licenses
listlengths 1
3
| version
stringclasses 677
values | tree_hash
stringlengths 40
40
| path
stringclasses 1
value | type
stringclasses 2
values | size
stringlengths 2
8
| text
stringlengths 25
67.1M
| package_name
stringlengths 2
41
| repo
stringlengths 33
86
|
---|---|---|---|---|---|---|---|---|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | code | 1073 | @safetestset "HTML+Julia rendering" begin
@safetestset "Simple tag rendering" begin
using Genie
using Genie.Renderer.Html
import Genie.Util: fws
copy = "Hello Genie"
@test Html.p(copy) |> fws == "<p>$copy</p>" |> fws
@test Html.div() |> fws == "<div></div>" |> fws
@test Html.br() |> fws == "<br$(Genie.config.html_parser_close_tag)>" |> fws
message = "Important message"
@test Html.span(message, class = "focus") |> fws ==
"<span class=\"focus\">$message</span>" |> fws
@test Html.span(message, class = "focus"; NamedTuple{(Symbol("data-process"),)}(("pre-process",))...) |> fws ==
"<span class=\"focus\" data-process=\"pre-process\">Important message</span>" |> fws
@test Html.span("Important message", class = "focus"; NamedTuple{(Symbol("data-process"),)}(("pre-process",))...) do
Html.a("Click here to read message")
end |> fws ==
"<span class=\"focus\" data-process=\"pre-process\" Important message><a>Click here to read message</a></span>" |> fws
end;
end; | Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | code | 2493 | @safetestset "HEAD requests" begin
@safetestset "HEAD requests should be by default handled by GET" begin
using Genie
using HTTP
port = nothing
port = rand(8500:8900)
route("/") do
"GET request"
end
server = up(port)
response = try
HTTP.request("GET", "http://127.0.0.1:$port", ["Content-Type" => "text/html"])
catch ex
ex.response
end
@test response.status == 200
@test String(response.body) == "GET request"
response = try
HTTP.request("HEAD", "http://127.0.0.1:$port", ["Content-Type" => "text/html"])
catch ex
ex.response
end
@test response.status == 200
@test String(response.body) == ""
down()
sleep(1)
server = nothing
port = nothing
end;
@safetestset "HEAD requests have no body" begin
using Genie
using HTTP
port = nothing
port = rand(8500:8900)
route("/") do
"Hello world"
end
route("/", method = HEAD) do
"Hello world"
end
server = up(port; open_browser = false)
response = try
HTTP.request("GET", "http://127.0.0.1:$port", ["Content-Type" => "text/html"])
catch ex
ex.response
end
@test response.status == 200
@test String(response.body) == "Hello world"
response = try
HTTP.request("HEAD", "http://127.0.0.1:$port", ["Content-Type" => "text/html"])
catch ex
ex.response
end
@test response.status == 200
@test isempty(String(response.body)) == true
down()
sleep(1)
server = nothing
port = nothing
end;
@safetestset "HEAD requests should overwrite GET" begin
using Genie
using HTTP
port = nothing
port = rand(8500:8900)
request_method = ""
route("/", named = :get_root) do
request_method = "GET"
"GET request"
end
route("/", method = "HEAD", named = :head_root) do
request_method = "HEAD"
"HEAD request"
end
server = up(port)
sleep(1)
response = try
HTTP.request("GET", "http://127.0.0.1:$port", ["Content-Type" => "text/html"])
catch ex
ex.response
end
@test response.status == 200
@test request_method == "GET"
response = try
HTTP.request("HEAD", "http://127.0.0.1:$port", ["Content-Type" => "text/html"])
catch ex
ex.response
end
@test response.status == 200
@test request_method == "HEAD"
down()
sleep(1)
server = nothing
port = nothing
end;
end;
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | code | 1199 | @safetestset "Fullstack app" begin
@safetestset "Create and run a full stack app with resources" begin
using Logging
Logging.global_logger(NullLogger())
testdir = pwd()
using Pkg
using Genie
content = "Test OK!"
workdir = Base.Filesystem.mktempdir()
cd(workdir)
Genie.Generator.newapp("fullstack_test", fullstack = true, testmode = true, interactive = false, autostart = false)
Genie.Generator.newcontroller("Foo", pluralize = false)
@test isfile(joinpath("app", "resources", "foo", "FooController.jl")) == true
mkpath(joinpath("app", "resources", "foo", "views"))
@test isdir(joinpath("app", "resources", "foo", "views")) == true
open(joinpath("app", "resources", "foo", "views", "foo.jl.html"), "w") do io
write(io, content)
end
@test isfile(joinpath("app", "resources", "foo", "views", "foo.jl.html")) == true
route("/test") do
Genie.Renderer.Html.html(:foo, :foo)
end
up(9999)
sleep(10)
r = Genie.Requests.HTTP.request("GET", "http://localhost:9999/test")
@test occursin(content, String(r.body)) == true
down()
sleep(1)
cd(testdir)
Pkg.activate(".")
end;
end; | Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | code | 1375 | @safetestset "DotEnv functionality" begin
@safetestset "ENV variables JSON endpoint check" begin
# using Logging
# Logging.global_logger(NullLogger())
# testdir = pwd()
# using Pkg
# using Genie
# tmpdir = Base.Filesystem.mktempdir()
# cd(tmpdir)
# @async Genie.Generator.newapp("testapp"; autostart=false, testmode=true)
# sleep(10)
# mv(".env.example", ".env")
# Genie.Loader.DotEnv.config()
# write("routes.jl",
# """
# using Genie.Router
# using Genie.Renderer.Json
# route("/") do
# Dict("PORT" => ENV["PORT"],
# "WSPORT" => ENV["WSPORT"],
# "HOST" => ENV["HOST"]) |> json
# end
# """)
# if Sys.iswindows()
# task = @async run(`bin\\server.bat`, wait=false)
# else
# task = @async run(`bin/server`, wait=false)
# end
# sleep(10)
# r = Genie.Requests.HTTP.request("GET", "http://$(ENV["HOST"]):$(ENV["PORT"])/")
# @test r.status == Genie.Router.OK
# eobj = Genie.Renderer.Json.JSONParser.parse(String(r.body))
# @test eobj["PORT"] == ENV["PORT"]
# @test eobj["WSPORT"] == ENV["WSPORT"]
# @test eobj["HOST"] == ENV["HOST"]
# try
# @async Base.throwto(task, InterruptException())
# catch
# end
# kill(task |> fetch)
# task = nothing
# cd(testdir)
# Pkg.activate(".")
end
end | Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | code | 705 | @safetestset "Sessions functionality" begin
@safetestset "Simple session setting and getting" begin
using Genie, Genie.Sessions
using Genie.Router
using HTTP
Genie.config.session_storage = :File
Sessions.init()
route("/home") do
sess = Sessions.session(params())
Sessions.set!(sess, :visit_count, Sessions.get(sess, :visit_count, 0)+1)
"$(Sessions.get(sess, :visit_count))"
end
server = up()
# TODO: extend to use the cookie and increment the count
response = HTTP.get("http://$(Genie.config.server_host):$(Genie.config.server_port)/home")
@test response.body |> String == "1"
down()
sleep(1)
server = nothing
end;
end; | Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | code | 154 | @safetestset "Sessions id test" begin
using Genie
@test !isempty(Genie.secret_token())
@test Genie.Sessions.id() != Genie.Sessions.id()
end;
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | code | 1082 | @safetestset "No Caching" begin
using Genie, Genie.Cache
Cache.init()
function f()
rand(1:1_000)
end
Genie.config.cache_duration = 0 # no caching
r0 = f()
r1 = withcache(:x) do
f()
end
@test r0 != r1 # because cache_duration == 0 so no caching
r2 = withcache(:x) do
f()
end
@test r1 != r2 # because cache_duration == 0 so no caching
end
@safetestset "cache" begin
using Genie, Genie.Cache
function f()
rand(1:1_000)
end
Genie.config.cache_duration = 5
r1 = withcache(:x) do
f()
end
r2 = withcache(:x) do
f()
end
@test r1 == r2
r3 = withcache(:x, condition = false) do # disable caching cause ! condition
f()
end
@test r1 == r2 != r3
r4 = withcache(:x, 0) do # disable caching with 0 duration
f()
end
@test r1 == r2 != r3 != r4
r5 = withcache(:x) do # regular cache should still work as under 5s passed
f()
end
@test r1 == r2 == r5
sleep(6)
r6 = withcache(:x) do # regular cache should not work as over 5s passed
f()
end
@test r1 == r2 == r5 != r6
end | Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | code | 317 | using Genie, Genie.Router, Genie.Renderer.Json
Genie.config.run_as_server = true
Genie.config.cors_allowed_origins = ["*"]
route("/random", method=POST) do
dim = parse(Int, get(params(), :dim, "2"))
num = parse(Int, get(params(), :num, "3"))
(:random => rand(dim,num)) |> json
end
up(; open_browser = false) | Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | code | 680 | using Pkg
Pkg.activate(".")
using Genie, Genie.Router, Genie.Renderer, Genie.Renderer.Html
form = """
<form action="/" method="POST" enctype="multipart/form-data">
<input type="text" name="greeting" value="hello genie" /><br/>
<input type="file" name="fileupload" /><br/>
<input type="submit" value="Submit" />
</form>
"""
route("/") do
html(form)
end
route("/", method = POST) do
for (name,file) in params(:FILES)
write(file.name, IOBuffer(file.data))
end
write("-" * params(:FILES)["fileupload"].name, IOBuffer(params(:FILES)["fileupload"].data))
@show params(:greeting)
params(:greeting)
end
Genie.Server.up(; open_browser = false, async = false)
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 5221 | # Contributor Covenant Code of Conduct
## Our Pledge
We as members, contributors, and leaders pledge to make participation in our
community a harassment-free experience for everyone, regardless of age, body
size, visible or invisible disability, ethnicity, sex characteristics, gender
identity and expression, level of experience, education, socio-economic status,
nationality, personal appearance, race, religion, or sexual identity
and orientation.
We pledge to act and interact in ways that contribute to an open, welcoming,
diverse, inclusive, and healthy community.
## Our Standards
Examples of behavior that contributes to a positive environment for our
community include:
* Demonstrating empathy and kindness toward other people
* Being respectful of differing opinions, viewpoints, and experiences
* Giving and gracefully accepting constructive feedback
* Accepting responsibility and apologizing to those affected by our mistakes,
and learning from the experience
* Focusing on what is best not just for us as individuals, but for the
overall community
Examples of unacceptable behavior include:
* The use of sexualized language or imagery, and sexual attention or
advances of any kind
* Trolling, insulting or derogatory comments, and personal or political attacks
* Public or private harassment
* Publishing others' private information, such as a physical or email
address, without their explicit permission
* Other conduct which could reasonably be considered inappropriate in a
professional setting
## Enforcement Responsibilities
Community leaders are responsible for clarifying and enforcing our standards of
acceptable behavior and will take appropriate and fair corrective action in
response to any behavior that they deem inappropriate, threatening, offensive,
or harmful.
Community leaders have the right and responsibility to remove, edit, or reject
comments, commits, code, wiki edits, issues, and other contributions that are
not aligned to this Code of Conduct, and will communicate reasons for moderation
decisions when appropriate.
## Scope
This Code of Conduct applies within all community spaces, and also applies when
an individual is officially representing the community in public spaces.
Examples of representing our community include using an official e-mail address,
posting via an official social media account, or acting as an appointed
representative at an online or offline event.
## Enforcement
Instances of abusive, harassing, or otherwise unacceptable behavior may be
reported to the community leaders responsible for enforcement at
[email protected].
All complaints will be reviewed and investigated promptly and fairly.
All community leaders are obligated to respect the privacy and security of the
reporter of any incident.
## Enforcement Guidelines
Community leaders will follow these Community Impact Guidelines in determining
the consequences for any action they deem in violation of this Code of Conduct:
### 1. Correction
**Community Impact**: Use of inappropriate language or other behavior deemed
unprofessional or unwelcome in the community.
**Consequence**: A private, written warning from community leaders, providing
clarity around the nature of the violation and an explanation of why the
behavior was inappropriate. A public apology may be requested.
### 2. Warning
**Community Impact**: A violation through a single incident or series
of actions.
**Consequence**: A warning with consequences for continued behavior. No
interaction with the people involved, including unsolicited interaction with
those enforcing the Code of Conduct, for a specified period of time. This
includes avoiding interactions in community spaces as well as external channels
like social media. Violating these terms may lead to a temporary or
permanent ban.
### 3. Temporary Ban
**Community Impact**: A serious violation of community standards, including
sustained inappropriate behavior.
**Consequence**: A temporary ban from any sort of interaction or public
communication with the community for a specified period of time. No public or
private interaction with the people involved, including unsolicited interaction
with those enforcing the Code of Conduct, is allowed during this period.
Violating these terms may lead to a permanent ban.
### 4. Permanent Ban
**Community Impact**: Demonstrating a pattern of violation of community
standards, including sustained inappropriate behavior, harassment of an
individual, or aggression toward or disparagement of classes of individuals.
**Consequence**: A permanent ban from any sort of public interaction within
the community.
## Attribution
This Code of Conduct is adapted from the [Contributor Covenant][homepage],
version 2.0, available at
https://www.contributor-covenant.org/version/2/0/code_of_conduct.html.
Community Impact Guidelines were inspired by [Mozilla's code of conduct
enforcement ladder](https://github.com/mozilla/diversity).
[homepage]: https://www.contributor-covenant.org
For answers to common questions about this code of conduct, see the FAQ at
https://www.contributor-covenant.org/faq. Translations are available at
https://www.contributor-covenant.org/translations.
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 1247 | # Contributing
When contributing to this repository, please first discuss the change you wish to make via `Issue`,
`Discord`, `Github Discussion`, with the owners of this repository before making a change. If it's a new feature the ideal place to discuss is [Genie Discussion](https://github.com/GenieFramework/Genie.jl/discussions)
Please note we have a code of conduct, please follow it in all your interactions with the project.
## Pull Request Process
1. Ensure any install or build dependencies are removed before the end of the layer when doing a
build.
2. Make sure you remove any OS generated configuration file like macos generates `.DS_Store` that stores custom attributes
3. Update the README.md with details of changes to the interface, this includes new environment
variables, exposed ports, useful file locations and container parameters.
4. Increase the version numbers in any examples files and the README.md to the new version that this
Pull Request would represent. The versioning scheme we use is [SemVer](https://semver.org).
5. You may merge the Pull Request once you have the sign-off of two other developers, or if you
do not have permission to do that, you may request the second reviewer merge it for you.
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 8202 | <div align="center">
<a href="https://genieframework.com/">
<img
src="docs/content/img/genie-lightblue.svg"
alt="Genie Logo"
height="64"
/>
</a>
<br />
<p>
<h3>
<b>
Genie.jl
</b>
</h3>
</p>
<p>
<b> 🧞 The highly productive Julia web framework
</b>
</p>
<p>
[](https://www.genieframework.com/docs/) [](https://github.com/GenieFramework/Genie.jl/blob/173d8e3deb47f20b3f8b4e5b12da6bf4c59f3370/Project.toml#L53) [](https://www.genieframework.com/) [](https://github.com/GenieFramework/Genie.jl/actions) [](https://pkgs.genieframework.com?packages=Genie) [](https://twitter.com/GenieMVC)
</p>
<p>
<sub>
Built with ❤︎ by
<a href="https://github.com/GenieFramework/Genie.jl/graphs/contributors">
contributors
</a>
</sub>
</p>
</div>
Genie.jl is the backbone of the [Genie Framework](https://genieframework.com), which provides a streamlined and efficient workflow for developing modern web applications. It builds on Julia's strengths (high-level, high-performance, dynamic, JIT compiled), exposing a rich API and a powerful toolset for productive web development.
Genie Framework is composed of four main components:
- **[Genie.jl](https://github.com/GenieFramework/Genie.jl)**: the server backend, providing features for routing, templating, authentication, and much more.
- **[Stipple.jl](https://github.com/GenieFramework/Stipple.jl)**: a package for building reactive UIs with a simple and powerful low-code API in pure Julia.
- **[Genie Builder](https://learn.genieframework.com/docs/genie-builder/quick-start)**: a VSCode plugin for building UIs visually in a drag-and-drop editor.
- **[SearchLight.jl](https://github.com/GenieFramework/SearchLight.jl)**: a complete ORM solution, enabling easy database integration without writing SQL queries.
To learn more about Genie, visit the [documentation](https://learn.genieframework.com/docs/guides), and the [app gallery](https://learn.genieframework.com/app-gallery).
If you need help with anything, you can find us on [Discord](https://discord.com/invite/9zyZbD6J7H).
https://github.com/GenieFramework/Genie.jl/assets/5058397/627dcda0-bb13-49f9-8827-2bfb581a9bb7
<p style="font-family:verdana;font-size:60%;margin-bottom:4%" align="center">
<u>Julia data dashboard powered by Genie. <a href="https://learn.genieframework.com/app-gallery">App gallery</a></u>
</p>
---
- [**Features of Genie.jl**](#features-of-genie.jl)
- [**Contributing**](#contributing)
- [**Special Credits**](#special-credits)
- [**License**](#license)
---
</details>
### **Features of Genie.jl**
🛠**Genie Router:** Genie has a really powerful
💪 `Router`. Matching web requests to functions, extracting and setting up the request's variables and the execution environment, and invoking the response methods. Features include:
- Static, Dynamic, Named routing
- Routing parameters
- Linking routes
- Route management (Listing, Deleting, Modifying) support
- Routing methods (`GET`, `POST`, `PUT`, `PATCH`, `DELETE`, `OPTIONS`)
- and more ...
```julia
# Genie Hello World!
# As simple as Hello
using Genie
route("/hello") do
"Welcome to Genie!"
end
# Powerful high-performance HTML view templates
using Genie.Renderer.Html
route("/html") do
h1("Welcome to Genie!") |> html
end
# JSON rendering built in
using Genie.Renderer.Json
route("/json") do
(:greeting => "Welcome to Genie!") |> json
end
# Start the app!
up(8888)
```
🔌 **WebSocket:** Genie provides a powerful workflow for client-server communication over websockets
```julia-repl
julia> using Genie, Genie.Router
julia> channel("/foo/bar") do
# process request
end
[WS] /foo/bar => #1 | :foo_bar
```
📃 **Templating:** Built-in templates support for `HTML`, `JSON`, `Markdown`, `JavaScript` views.
🔐 **Authentication:** Easy to add database backed authentication for restricted area of a website.
```julia-repl
julia> using Pkg
julia> Pkg.add("GenieAuthentication") # adding authentication plugin
julia> using GenieAuthentication
julia> GenieAuthentication.install(@__DIR__)
```
⏰ **Tasks:** Tasks allow you to perform various operations and hook them with crons jobs for automation
```julia
module S3DBTask
# ... hidden code
"""
Downloads S3 files to local disk.
Populate the database from CSV file
"""
function runtask()
mktempdir() do directory
@info "Path of directory" directory
# download record file
download(RECORD_URL)
# unzip file
unzip(directory)
# dump to database
dbdump(directory)
end
end
# ... more hidden code
end
```
```shell
$ bin/runtask S3DBTask
```
📦 **Plugin Ecosystem:** Explore plugins built by the community such as [GenieAuthentication](https://github.com/GenieFramework/GenieAuthentication.jl), [GenieAutoreload](https://github.com/GenieFramework/GenieAutoreload.jl), [GenieAuthorisation](https://github.com/GenieFramework/GenieAuthorisation.jl), and more
🗃️ **ORM Support:** Explore [SearchLight](https://github.com/GenieFramework/SearchLight.jl) a complete ORM solution for Genie, supporting Postgres, MySQL, SQLite and other adapters
```julia
function search(user_names, regions, startdate, enddate)
# ... hidden code
where_filters = SQLWhereEntity[
SQLWhereExpression("lower(user_name) IN ( $(repeat("?,", length(user_names))[1:end-1] ) )", user_names),
SQLWhereExpression("date >= ? AND date <= ?", startdate, enddate)
]
SearchLight.find(UserRecord, where_filters, order=["record.date"])
# ... more hidden code
end
```
- `Database Migrations`
```julia
module CreateTableRecord
import SearchLight.Migrations: create_table, column, primary_key, add_index, drop_table
function up()
create_table(:record) do
[
primary_key()
column(:user_uuid, :string, limit = 100)
column(:user_name, :string, limit = 100)
column(:status, :integer, limit = 4)
column(:region, :string, limit = 20)
column(:date_of_birth, :string, limit = 100)
]
end
add_index(:record, :user_uuid)
add_index(:record, :user_name)
add_index(:record, :region)
add_index(:record, :date_of_birth)
end
function down()
drop_table(:record)
end
end
```
* `Model Validations`
📝 More Genie features like:
* `Files Uploads`
```julia
route("/", method = POST) do
if infilespayload(:yourfile)
write(filespayload(:yourfile))
stat(filename(filespayload(:yourfile)))
else
"No file uploaded"
end
end
```
* `Logging` | `Caching` | `Cookies and Sessions` | `Docker, Heroku, JuliaHub, etc Integrations` | `Genie Deploy`
* To explore more features check [Genie Documentation](https://www.genieframework.com/docs/genie/tutorials/Overview.html) 🏃♂️🏃♀️
## **Contributing**
Please contribute using [GitHub Flow](https://guides.github.com/introduction/flow). Create a branch, add commits, and [open a pull request](https://github.com/genieframework/genie.jl/compare).
Please read [`CONTRIBUTING`](CONTRIBUTING.md) for details on our [`CODE OF CONDUCT`](CODE_OF_CONDUCT.md), and the process for submitting pull requests to us.
## **Special Credits**
* The awesome Genie logo was designed by Alvaro Casanova
* Hoppscoth for readme structure template
* Genie uses a multitude of packages that have been kindly contributed by the Julia community
## **License**
This project is licensed under the [MIT License](https://opensource.org/licenses/MIT) - see the [`LICENSE`](https://github.com/GenieFramework/Genie.jl/blob/master/LICENSE.md) file for details.
<p>⭐ If you enjoy this project please consider starring the 🧞 <b>Genie.jl</b> GitHub repo. It will help us fund our open source projects.</p>
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 2457 | # Security Policy
## Supported Versions
Versions of Genie.jl currently being supported with security updates.
| Version | Supported |
| ---------- | ------------------ |
| >= 4.16.x | ✔ |
| <= 4.15.x | x |
# Security Policies and Procedures
This document outlines security procedures and general policies for the Genie
project.
* [Reporting a Bug](#reporting-a-bug)
* [Disclosure Policy](#disclosure-policy)
* [Comments on this Policy](#comments-on-this-policy)
## Reporting a Bug
The Genie team and community take all security bugs in Genie seriously.
Thank you for improving the security of Genie. We appreciate your efforts and
responsible disclosure and will make every effort to acknowledge your
contributions.
Report security bugs by emailing the lead maintainer on following email: <[email protected]> with subject as followed:
`**[SECURITY Genie.jl] followed with subject of security bug**`
To ensure the timely response to your report, please ensure that the entirety
of the report is contained within the email body and not solely behind a web
link or an attachment.
The lead maintainer will acknowledge your email within 42 hours, and will send a
more detailed response within 42 hours indicating the next steps in handling
your report. After the initial reply to your report, the security team will
endeavor to keep you informed of the progress towards a fix and full
announcement, and may ask for additional information or guidance.
Report security bugs in third-party modules to the person or team maintaining
the module with cc'ed [email protected] if Genie.jl is using the module as one of the dependencies
## Disclosure Policy
When the security team receives a security bug report, they will assign it to a
primary handler. This person will coordinate the fix and release process,
involving the following steps:
* Confirm the problem and determine the affected versions.
* Audit code to find any potential similar problems.
* Prepare fixes for all releases still under maintenance. These fixes will be
released as fast as possible and will be available with latest tagged Genie version and announced on [Genie's twitter](https://twitter.com/GenieMVC) and [Genie's Discord #announcement channel](https://discord.com/invite/9zyZbD6J7H).
## Comments on this Policy
If you have suggestions on how this process could be improved please submit a
pull request.
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 779 | ---
name: Bug report
about: Report Genie issues
title: ''
labels: ''
assignees: essenciary
---
**Describe the bug**
A clear and concise description of what the bug is.
**Error stacktrace**
If any error is thrown, please copy from the REPL and paste it here
**To reproduce**
Steps to reproduce the behavior and/or Julia code executed.
**Expected behavior**
A clear and concise description of what you expected to happen.
**Additional context**
Please include the output of
`julia> versioninfo()`
and
`pkg> st`
---
**Please answer these optional questions to help us understand, prioritise, and assign the issue**
1/ Are you using Genie at work or for hobby/personal projects?
2/ Can you give us, in a few words, some details about the app you're building with Genie?
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 595 | ---
name: Feature request
about: Suggest an idea for this project
title: ''
labels: ''
assignees: ''
---
**Is your feature request related to a problem? Please describe.**
A clear and concise description of what the problem is. Ex. I'm always frustrated when [...]
**Describe the solution you'd like**
A clear and concise description of what you want to happen.
**Describe alternatives you've considered**
A clear and concise description of any alternative solutions or features you've considered.
**Additional context**
Add any other context or screenshots about the feature request here.
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 786 | # Genie
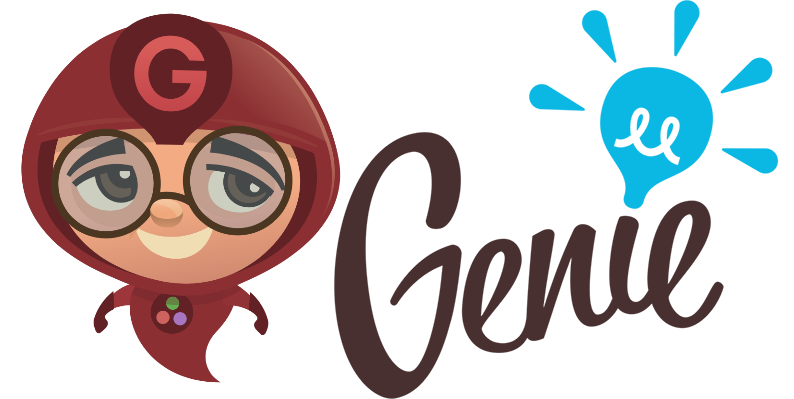
# Genie
## The highly productive Julia web framework
Genie is a full-stack MVC web framework that provides a streamlined and efficient workflow for developing modern web applications. It builds on Julia's strengths (high-level, high-performance, dynamic, JIT compiled), exposing a rich API and a powerful toolset for productive web development.
### Current status
Genie is compatible with Julia v1.6 and up.
---
## Documentation
<https://genieframework.github.io/Genie.jl/dev/>
---
## Acknowledgements
* Genie uses a multitude of packages that have been kindly contributed by the Julia community.
* The awesome Genie logo was designed by Alvaro Casanova.
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 497 | ```@meta
CurrentModule = Assets
```
```@docs
add_fileroute
AssetsConfig
assets_config!
assets_endpoint
asset_file
asset_path
asset_route
channels
channels_route
channels_script
channels_script_tag
channels_subscribe
channels_support
css_asset
embedded
embedded_path
external_assets
favicon_support
include_asset
js_asset
jsliteral
js_settings
webthreads
webthreads_endpoint
webthreads_push_pull
webthreads_route
webthreads_script
webthreads_script_tag
webthreads_subscribe
webthreads_support
```
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 98 | ```@meta
CurrentModule = Commands
```
```@docs
called_command
execute
parse_commandline_args
```
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 116 | ```@meta
CurrentModule = Configuration
```
```@docs
buildpath
config!
env
isdev
isprod
istest
pkginfo
Settings
```
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 98 | ```@meta
CurrentModule = Cookies
```
```@docs
Cookies.Dict
get
getcookies
set!
nullablevalue
```
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 87 | ```@meta
CurrentModule = Encryption
```
```@docs
decrypt
encrypt
encryption_sauce
```
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 153 | ```@meta
CurrentModule = Exceptions
```
```@docs
ExceptionalResponse
FileExistsException
InternalServerException
NotFoundException
RuntimeException
```
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 97 | ```@meta
CurrentModule = FileTemplates
```
```@docs
appmodule
newcontroller
newtask
newtest
```
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 637 | ```@meta
CurrentModule = Generator
```
```@docs
autoconfdb
autostart_app
binfolderpath
controller_file_name
db_intializer
db_support
fullstack_app
generate_project
install_app_dependencies
install_db_dependencies
install_searchlight_dependencies
microstack_app
minimal
mvc_support
newapp
newapp_fullstack
newapp_mvc
newapp_webservice
newcontroller
newresource
newtask
pkggenfile
pkgproject
post_create
remove_searchlight_initializer
resource_does_not_exist
scaffold
set_files_mod
setup_resource_path
setup_nix_bin_files
setup_windows_bin_files
validname
write_app_custom_files
write_db_config
write_resource_file
write_secrets_file
```
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 104 | ```@meta
CurrentModule = Genie
```
```@docs
bootstrap
down
down!
genie
go
isrunning
loadapp
run
up
```
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 176 | ```@meta
CurrentModule = Genie.Headers
```
```@docs
normalize_header_key
normalize_headers
set_access_control_allow_headers!
set_access_control_allow_origin!
set_headers!
```
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 68 | ```@meta
CurrentModule = HTTPUtils
```
```@docs
HTTPUtils.Dict
```
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 666 | 
# Genie
## The highly productive Julia web framework
Genie is Julia web framework that provides a streamlined and efficient workflow for developing modern web applications. It builds on Julia's strengths (high-level, high-performance, dynamic, JIT compiled), exposing a rich API and a powerful toolset for productive web development.
### Current status
Genie is compatible with Julia v1.3 and up.
---
## Acknowledgements
* Genie uses a multitude of packages that have been kindly contributed by the Julia community.
* The awesome Genie logo was designed by Alvaro Casanova.
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 212 | ```@meta
CurrentModule = Input
```
```@docs
HttpFile
HttpFormPart
HttpInput
all
files
get_multiform_parts!
parse_seicolon_fields
post
post_from_request!
parse_quoted_params
post_multipart!
post_url_encoded!
```
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 181 | ```@meta
CurrentModule = Loader
```
```@docs
autoload
bootstrap
default_context
importenv
load
load_helpers
load_initializers
load_libs
load_plugins
load_resources
load_routes
```
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 86 | ```@meta
CurrentModule = Logger
```
```@docs
initialize_logging
timestamp_logger
```
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 561 | ```@meta
CurrentModule = Renderer.Html
```
```@docs
normal_element
prepare_template
attributes
parseattr
normalize_element
denormalize_element
void_element
skip_element
include_markdown
get_template
doctype
doc
parseview
render
parsehtml
Genie.Renderer.render
html
safe_attr
parsehtml
html_to_julia
string_to_julia
to_julia
partial
template
read_template_file
parse_template
parse_string
parse
parsetags
register_elements
register_element
register_normal_element
register_void_element
attr
for_each
collection
Genie.Router.error
serve_error_file
@yield
el
```
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 103 | ```@meta
CurrentModule = Renderer.Js
```
```@docs
get_template
to_js
render
js
Genie.Router.error
```
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 110 | ```@meta
CurrentModule = Renderer.Json
```
```@docs
render
Genie.Renderer.render
json
Genie.Router.error
```
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 286 | ```@meta
CurrentModule = Renderer
```
```@docs
WebRenderable
render
redirect
hasrequested
respond
registervars
injectvars
view_file_info
vars_signature
function_name
m_name
build_is_stale
build_module
preparebuilds
purgebuilds
changebuilds
set_negotiated_content
negotiate_content
```
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 217 | ```@meta
CurrentModule = Requests
```
```@docs
jsonpayload
rawpayload
filespayload
infilespayload
Requests.write
Requests.read
filename
postpayload
getpayload
request
payload
matchedroute
matchedchannel
wsclient
```
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 155 | ```@meta
CurrentModule = Responses
```
```@docs
getresponse
getheaders
setheaders!
setheaders
getstatus
setstatus!
setstatus
getbody
setbody!
setbody
```
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 804 | ```@meta
CurrentModule = Router
```
```@docs
Route
Channel
Router.show
Params
ispayload
route_request
route_ws_request
Router.push!
route
channel
routename
channelname
baptizer
named_routes
routes
named_channels
channels
get_route
routes
channels
delete!
to_link
tolink
link_to
linkto
toroute
route_params_to_dict
action_controller_params
run_hook
match_routes
match_channels
parse_route
parse_channel
extract_uri_params
extract_get_params
extract_post_params
extract_request_params
content_type
content_length
request_type_is
request_type
nested_keys
setup_base_params
to_response
params
_params_
request
response_type
append_to_routes_file
is_static_file
to_uri
escape_resource_path
serve_static_file
preflight_response
response_mime
file_path
filepath
pathify
file_extension
file_headers
ormatch
```
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 90 | ```@meta
CurrentModule = Secrets
```
```@docs
load
secret
secret_token
secret_token!
```
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 256 | ```@meta
CurrentModule = Server
```
```@docs
SERVERS
ServersCollection
down
down!
handle_request
handle_ws_request
isrunning
openbrowser
print_server_status
serve
server_status
setup_http_listener
setup_http_streamer
setup_ws_handler
up
update_config
```
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 117 | ```@meta
CurrentModule = Sessions
```
```@docs
Session
id
start
set!
get
unset!
isset
persist
load
session
init
```
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 181 | ```@meta
CurrentModule = Toolbox
```
```@docs
TaskInfo
TaskResult
tasks
VoidTaskResult
validtaskname
taskdocs
loadtasks
printtasks
new
taskfilename
taskmodulename
isvalidtask!
```
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 123 | ```@meta
CurrentModule = Util
```
```@docs
expand_nullable
file_name_without_extension
walk_dir
time_to_unixtimestamp
```
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 129 | ```@meta
CurrentModule = Watch
```
```@docs
WATCHED_FOLDERS
WATCHING
collect_watched_files
handlers
unwatch
watch
watchpath
```
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 409 | ```@meta
CurrentModule = WebChannels
```
```@docs
ChannelNotFoundException
ChannelClient
ChannelClientsCollection
ChannelSubscriptionsCollection
MessagePayload
ChannelMessage
CLIENTS
SUBSCRIPTIONS
clients
subscriptions
websockets
channels
connected_clients
disconnected_clients
subscribe
unsubscribe
unsubscribe_client
unsubscribe_disconnected_clients
push_subscription
pop_subscription
broadcast
message
``` | Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 466 | ```@meta
CurrentModule = WebThreads
```
```@docs
CLIENTS
MESSAGE_QUEUE
SUBSCRIPTIONS
ChannelClient
ChannelClientsCollection
ChannelMessage
ChannelSubscriptionsCollection
ClientId
ChannelName
MessagePayload
broadcast
channels
clients
connected_clients
disconnected_clients
message
pop_subscription
pull
push
push_subscription
subscribe
subscriptions
timestamp_client
unsubscribe
unsubscribe_client
unsubscribe_clients
unsubscribe_disconnected_clients
webthreads
```
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 1213 | # Controlling load order of julia files in Genie Apps
The default load sequence of julia files in Genie Apps is alphabetical given any directory inside your genie app `plugins`, `libs`, `controllers`. It looks like below:
```
Aab.jl
Abb.jl
Dde.jl
Zyx.jl
```
But sometimes user wants to control the default load order of `.jl` files inside Genie Apps
### Two ways to control load order in Genie Apps
#### 1) `.autoload_ignore`:
Creating an empty `.autoload_ignore` file in a directory causes all files contained in the folder to be excluded from Genie's startup load order.
#### 2) `.autoload`
Let's assume we have a directory with a few of `.jl` files as follows
```
Aaa.jl
Abb.jl
Abc.jl
def.jl
Foo.jl
-x-yz.jl
```
and we want to decide the load order of these files. To achieve this behavior, we can simply create a `.autoload` file in the directory containing your `.jl` files with content(developer preferred load order) as follows:
```
--x-yz.jl
def.jl
Abc.jl
-Foo.jl
```
where (-) means exclude the file from Genie's autoload sequence and (--) means remove file starting with (-) from Genie Load sequence. Now the load order of our directory is going to be
```
def.jl
Abc.jl
Aaa.jl
Abb.jl
```
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 3513 | ### How to deploy Genie applications
In this guide we will walk through how to deploy a Genie App to Amazon Cloud. This article will be added with more information in future.
### Prerequisite:
* Genie Application with ready Dockerfile(Refer to Genie Docker Tutorial)
* Opened account in main cloud providers
That’s it, from this point we can start with deployment.
## AWS
### Networking
The easiest and quickest to deploy and expose Genie applications is to create a EC2 instance and run docker container inside it.
After opening an AWS console you should be able to see your network under VPC tab. This is default one, and we’ll use it for hosting our EC2 instance.

This VPC already contains a predefined subnets. We need a public subnet as we should access our Application via Internet.

Great, let’s move on.
### Setup EC2 instance
Navigate to EC2 tab in AWS Console and then click on “Launch instances“.

We will use a Free Tier Amazon Linux 2 AMI image. It can fit our needs for this demo

For an Instance type lets choose t2.small, in that case we’ll get 1vCPU and 2Gb Memory. We should have enough memory with this instance to meet our application requirements. Also we should allow HTTP & HTTPS access. For SSH let’s specify your IP or let 0.0.0.0/0 with is allows connect to this instance from everywhere (not secure). Also double check that “Auto-assign public IP“ is enabled.

Click on "Launch instance". It’ll take around 2min and we can proceed.

Ok, now our Instance is Running and have a public IP.
### Setup Docker on EC2
Now let’s connect to is and install a docker. Here is the command to connect via SSH. Please change this IP by yours.
```
ssh -i my-demo-app.pem [email protected]
```
Let’s Install Docker on it, it’s pretty easy, just execute next commands:
```
sudo yum update
sudo yum install docker -y
sudo usermod -a -G docker ec2-user
id ec2-user
newgrp docker
sudo systemctl enable docker.service
sudo systemctl start docker.service
```
Now we can verify that our docker was installed and runs successfully
```
sudo systemctl status docker.service
```
For more information you can check this [guide](https://www.cyberciti.biz/faq/how-to-install-docker-on-amazon-linux-2/). Amazing, basically that’s it, now we can deploy our application.
Let’s clone repository with our applications and Dockerfile to EC2. If git is not installed on EC2 just execute
```
sudo yum git install -y
# And clone your git repo
git clone <your-repo.git>
cd <your-repo>
```
### Building a Genie Application
First of all let’s build our app using docker cli
```
docker build -t my-demo-app .
docker images
```
If build was successful your should be able to see docker image. Now let’s just start it. In our case application works on port 8000, so we did a port-mapping to port 80.
```
docker run -p 80:8000 my-demo-app
```
Then just grep a public IP from AWS console and open it in your browser.

That’s it, your application was successfully deployed. Thanks.
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 6155 | # Genie Plugins
Genie plugins are special Julia packages which extend Genie apps with powerful functionality by providing specific integration points. A Genie plugin is made of two parts:
1. the Julia package exposing the core functionality of the plugin, and
2. a files payload (controllers, modules, views, database migrations, initializers, etc) which are copied into the client app upon plugin installation.
## Using Genie Plugins
The plugins are created by third party Genie/Julia developers. Take this simple demo plugin as an example: <https://github.com/GenieFramework/HelloPlugin.jl>
In order to add the plugin to an existing Genie app you need to:
Add the `HelloPlugin` package to your Genie app, just like any other Julia Pkg dependency:
```julia
pkg> add https://github.com/GenieFramework/HelloPlugin.jl
```
Bring the package into scope:
```julia
julia> using HelloPlugin
```
Install the plugin (this is a one time operation, when the package is added):
```julia
julia> HelloPlugin.install(@__DIR__)
```
### Running the Plugin
The installation will add a new `hello` resource in `app/resources/hello/` in the form of `HelloController.jl` and `views/greet.jl.html`. Also, in your Genie app's `plugins/` folder you fill find a new file, `helloplugin.jl` (which is the plugins' initializer and is automatically loaded by Genie early in the bootstrap process).
The `helloplugin.jl` initializer is defining a new route `route("/hello", HelloController.greet)`. If you restart your Genie app and navigate to `/hello` you will get the plugin's greeting.
## Walkthrough
Create a new Genie app:
```julia
julia> using Genie
julia> Genie.Generator.newapp("Greetings", autostart = false)
```
Add the plugin as a dependency:
```julia
julia> ]
pkg> add https://github.com/GenieFramework/HelloPlugin.jl
```
Bring the plugin into scope and run the installer (the installer should be run only once, upon adding the plugin package)
```julia
julia> using HelloPlugin
julia> HelloPlugin.install(@__DIR__)
```
The installation might show a series of logging messages informing about failure to copy some files or create folders. Normally it's nothing to worry about: these are due to the fact that some of the files and folders already exist in the app so they are not overwritten by the installer.
Restart the app to load the plugin:
```julia
julia> exit()
$ cd Greetings/
$ bin/repl
```
Start the server:
```julia
julia> up()
```
Navigate to `http://localhost:8000/hello` to get the greeting from the plugin.
---
## Developing Genie Plugins
`GeniePlugins.jl` is a Genie plugin that provides an efficient scaffold for creating a new Genie plugin packages. All you need to do is run this code to create your plugin project:
```julia
julia> using Genie, GeniePlugins
julia> GeniePlugins.scaffold("GenieHelloPlugin") # use the actual name of your plugin
Generating project file
Generating project GenieHelloPlugin:
GenieHelloPlugin/Project.toml
GenieHelloPlugin/src/GenieHelloPlugin.jl
Scaffolding file structure
Adding dependencies
Updating registry at `~/.julia/registries/General`
Updating git-repo `https://github.com/JuliaRegistries/General.git`
Updating git-repo `https://github.com/genieframework/Genie.jl`
Resolving package versions...
# output truncated
Initialized empty Git repository in /Users/adrian/GenieHelloPlugin/.git/
[master (root-commit) 30533f9] initial commit
11 files changed, 261 insertions(+)
Congratulations, your plugin is ready!
You can use this default installation function in your plugin's module:
function install(dest::String; force = false)
src = abspath(normpath(joinpath(@__DIR__, "..", GeniePlugins.FILES_FOLDER)))
for f in readdir(src)
isdir(f) || continue
GeniePlugins.install(joinpath(src, f), dest, force = force)
end
end
```
The scaffold command will create the file structure of your plugin, including the Julia project, the `.git` repo, and the file structure for integrating with Genie apps:
```
.
├── Manifest.toml
├── Project.toml
├── files
│ ├── app
│ │ ├── assets
│ │ │ ├── css
│ │ │ ├── fonts
│ │ │ └── js
│ │ ├── helpers
│ │ ├── layouts
│ │ └── resources
│ ├── db
│ │ ├── migrations
│ │ └── seeds
│ ├── lib
│ ├── plugins
│ │ └── geniehelloplugin.jl
│ └── task
└── src
└── GenieHelloPlugin.jl
```
The core of the functionality should go into the `src/GenieHelloPlugin.jl` module. While everything placed within the `files/` folder should be copied into the corresponding folders of the Genie apps installing the plugin. You can add resources, controllers, models, database migrations, views, assets and any other files inside the `files/` folder to be copied.
The scaffolding will also create a `plugins/geniehelloplugin.jl` file - this is the initializer of the plugin and is meant to bootstrap the functionality of the plugin. Here you can load dependencies, define routes, set up configuration, etc.
Because any Genie plugin is a Julia `Pkg` project, you can add any other Julia packages as dependencies.
### The Installation Function
The main module file, present in `src/GenieHelloPlugin.jl` should also expose an `install(path::String)` function, responsible for copying the files of your plugin into the user Genie app. The `path` param is the root of the Genie app where the installation will be performed.
As copying the plugin's files is a standard but tedious operation, Genie provides some helpers to get you started. The `GeniePlugins` module provides an `install(path::String, dest::String; force = false)` which can be used for copying the plugin's files to their destination in the app.
The scaffolding function will also recommend a default `install(path::String)` that you can use in your module:
```julia
function install(dest::String; force = false)
src = abspath(normpath(joinpath(@__DIR__, "..", GeniePlugins.FILES_FOLDER)))
for f in readdir(src)
isdir(f) || continue
GeniePlugins.install(joinpath(src, f), dest, force = force)
end
end
```
You can use it as a starting point - and add any other specific extra logic to it.
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 2223 | # Using Genie in an interactive environment (Jupyter/IJulia, REPL, etc)
Genie can be used for ad-hoc exploratory programming, to quickly whip up a web server
and expose your Julia functions.
Once you have `Genie` into scope, you can define a new `route`.
A `route` maps a URL to a function.
```julia
julia> using Genie
julia> route("/") do
"Hi there!"
end
```
You can now start the web server using
```julia
julia> up()
```
Finally, now navigate to <http://localhost:8000> – you should see the message "Hi there!".
We can define more complex URIs which can also map to previously defined functions:
```julia
julia> function hello_world()
"Hello World!"
end
julia> route("/hello/world", hello_world)
```
The route handler functions can be defined anywhere (in any other file or module) as long as they are accessible in the current scope.
You can now visit <http://localhost:8000/hello/world> in the browser.
We can access route params that are defined as part of the URL, like `:message` in the following example:
```julia
julia> route("/echo/:message") do
params(:message)
end
```
Accessing <http://localhost:8000/echo/ciao> should echo "ciao".
And we can even match route params by types (and automatically convert them to the correct type):
```julia
julia> route("/sum/:x::Int/:y::Int") do
params(:x) + params(:y)
end
```
By default, route params are extracted as `SubString` (more exactly, `SubString{String}`).
If type constraints are added, Genie will attempt to convert the `SubString` to the indicated type.
For the above to work, we also need to tell Genie how to perform the conversion:
```julia
julia> Base.convert(::Type{Int}, s::AbstractString) = parse(Int, s)
```
Now if we access <http://localhost:8000/sum/2/3> we should see `5`
## Handling query params
Query params, which look like `...?foo=bar&baz=2` are automatically unpacked by Genie and placed into the `params` collection. For example:
```julia
julia> route("/sum/:x::Int/:y::Int") do
params(:x) + params(:y) + parse(Int, get(params, :initial_value, "0"))
end
```
Accessing <http://localhost:8000/sum/2/3?initial_value=10> will now output `15`.
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 10188 | # Migrating Genie apps from v4 to v5
Genie v5 is a major update to the Genie framework that introduces many new features and improvements. These include a nimble core architecture with many previously bundled features being now moved to stand-alone packages (cache, deployment, etc), the removal of legacy code and APIs, performance refactoring, restructuring of the core framework and APIs, and many other reliability, usability and performance improvements.
## Support for Genie v4
Despite introducing breaking changes, the upgrade from Genie v4 to v5 should be quite straightforward and should not take a long time. All users are recommended to upgrade to Genie v5.
While we will not backport compatible features from Genie 5 to Genie 4, we will continue to support the v4 API for the foreseeable period of time and we will backport compatible security patches.
### Genie v4 and Julia v1.8 compatibility issue: `modules_warned_for not defined`
Due to an issue caused by the removal of some APIs in Julia 1.8, **Genie v4 apps can not run on Julia 1.8**. This results in a `LoadError: UndefVarError: modules_warned_for not defined` exception when loading a Genie v4 app on Julia 1.8 and above.
### Addressing the issue: `modules_warned_for not defined`
The obvious and immediate solution is to simply go ahead and comment out the offending line -- the exact line will depend on your specific app, but it's line 4 in `bootstrap.jl` and starts with `push!(Base.modules_warned_for, Base.PkgId`.
However, this only eliminates the source of the exception. But it's possible that the Genie v4 app will still not run on Julia 1.8 and above as is, due to the fact that the loading of resources (controllers and models) in the app no longer works. So if the app does not work, mainly due to exceptions mentioning that the app does not have some controller or model "in its dependencies", this is the reason. The only way to fix this is to upgrade to Genie v5 and update your app to support Genie v5 by following the following steps.
## Upgrade from v4 to v5
However, some of these deep changes come at the cost of breaking compatibility with older apps that were developed using Genie v4. This guide will walk you through the process of migrating a Genie app from v4 to v5. The following changes need to be made to various Genie v4 application files. Each section indicates the file that needs to be modified.
### 1. `config/secrets.jl`
In Genie v5, `Genie.secret_token!` has been moved to a dedicated module called `Secrets`, so the `config/secrets.jl` file needs to be updated to include the new module.
``` julia
Genie.Secrets.secret_token!("<your-secret-token>")
```
### 2. `config/initializers/ssl.jl`
Genie v5 completely removed the legacy `config/initializers/ssl.jl` file. This provided a crude way of setting up SSL support for local development that was limited and not reliable. In Genie v5 the recommended approach is to set up SSL at the proxy server level, for example by using Caddy, Nginx, or Apache as a reverse proxy.
So just remove the `config/initializers/ssl.jl` file.
```julia
julia> rm("config/initializers/ssl.jl")
```
### 3. `app/helpers/ViewHelper.jl`
The `output_flash` function defined in the `ViewHelper` file uses the `flash` function which relied on `Genie.Session` in Genie v4. In Genie v5, `Genie.Session` has been moved to a dedicated plugin called `GenieSession.jl`. In addition, `GenieSession.jl` is designed to support multiple backends for session storage (file system, database, etc) so we also need to add a backend, such as `GenieSessionFileSession.jl`.
If your app uses the `output_flash` function then you need to add `GenieSession.jl` as a dependency of your app and update the `ViewHelper` file to use the `GenieSession.Flash.flash` function.
```julia
module ViewHelper
using GenieSession, GenieSessionFileSession, GenieSession.Flash
export output_flash
function output_flash(flashtype::String = "danger") :: String
flash_has_message() ? """<div class="alert alert-$flashtype alert-dismissable">$(flash())</div>""" : ""
end
end
```
If your app does not use `output_flash` then you can just remove the `ViewHelper` file.
```julia
julia> rm("app/helpers/ViewHelper.jl")
```
### 4. All references to app resources (controllers and models)
In Genie v5, the app resources such as controllers and models were accessible directly in `routes.jl` and in any other resource. For instance, let's say that we have:
* a controller called `HomeController`
* a model called `Home`
* our app (aka our project's main module) is called `WelcomeHome` (meaning that we have a file `src/WelcomeHome.jl`)
In v4 we could access them directly in `routes.jl` (or in other controllers and models) as `using HomeController, Home`.
However, in Genie v5 all the app's resources are scoped to the app's main module. So we need to update all references to the app resources to use the app's main module, meaning that in v5 we'll need `using WelcomeHome.HomeController, WelcomeHome.Home`.
We can also dynamically reference the app's main module by using `..Main.UserApp` (so `..Main.UserApp` is the same as `WelcomeHome` in our example).
### 5. `Genie.Cache`
In Genie v5, the `Genie.Cache` module has been moved to a dedicated plugin called `GenieCache.jl`. This means that all references to `Genie.Cache` need to be updated to use `GenieCache`.
This change was made to provide a leaner Genie core, making caching features opt-in. But also to allow the independent development of the caching features, independent from Genie itself.
### 6. `Genie.Cache.FileCache`
Starting with Genie 5, the file-based caching functionality provided by `Genie.Cache.FileCache` has been moved to a dedicated plugin called `GenieCacheFileCache.jl`. This means that all references to `Genie.Cache.FileCache` need to be updated to use `GenieCacheFileCache`. The `GenieCacheFileCache` plugin is dependent on the `GenieCache` package and it extends the functionality of `GenieCache`.
In the future, additional cache backends will be released.
### 7. `Genie.Session`
As mentioned above, the `Genie.Session` module has been moved to a dedicated plugin called `GenieSession.jl`. This means that all references to `Genie.Session` need to be updated to use `GenieSession`.
This change was made to provide a leaner Genie core, making session related features opt-in. But also to allow the independent development of the session features, independent from Genie itself.
### 8. `Genie.Session.FileSession`
Starting with Genie 5, the file-based session storage provided by `Genie.Session.FileSession` has been moved to a dedicated plugin called `GenieSessionFileSession.jl`. This means that all references to `Genie.Session.FileSession` need to be updated to use `GenieSessionFileSession`. The `GenieSessionFileSession` plugin is dependent on the `GenieSession` package and it extends the functionality of `GenieSession`.
In the future, additional session storage backends will be released.
### 9. `Genie.Deploy`
Similar to `Genie.Session` and `Genie.Cache`, the `Genie.Deploy` module has been moved to a dedicated plugin called `GenieDeploy.jl`. This means that all references to `Genie.Deploy` need to be updated to use `GenieDeploy`.
### 10. `Genie.Deploy.Docker`
Starting with Genie 5, the `Docker` deployment functionality provided by `Genie.Deploy.Docker` has been moved to a dedicated plugin called `GenieDeployDocker.jl`. This means that all references to `Genie.Deploy.Docker` need to be updated to use `GenieDeployDocker`. The `GenieDeployDocker` plugin is dependent on the `GenieDeploy` package and it extends the functionality of `GenieDeploy`.
### 11. `Genie.Deploy.Heroku`
Starting with Genie 5, the `Heroku` deployment functionality provided by `Genie.Deploy.Heroku` has been moved to a dedicated plugin called `GenieDeployHeroku.jl`. This means that all references to `Genie.Deploy.Heroku` need to be updated to use `GenieDeployHeroku`. The `GenieDeployHeroku` plugin is dependent on the `GenieDeploy` package and it extends the functionality of `GenieDeploy`.
### 12. `Genie.Deploy.JuliaHub`
Starting with Genie 5, the `JuliaHub` deployment functionality provided by `Genie.Deploy.JuliaHub` has been moved to a dedicated plugin called `GenieDeployJuliaHub.jl`. This means that all references to `Genie.Deploy.JuliaHub` need to be updated to use `GenieDeployJuliaHub`. The `GenieDeployJuliaHub` plugin is dependent on the `GenieDeploy` package and it extends the functionality of `GenieDeploy`.
### 13. `Genie.App`
The `Genie.App` module has been removed in v5 and its API has been moved to the `Genie` module.
### 14. `Genie.AppServer`
The `Genie.AppServer` module has been renamed to `Genie.Server` in v5.
### 15. `Genie.Plugins`
Starting with Genie 5, the `Genie.Plugins` functionality has been moved to a dedicated plugin called `GeniePlugins.jl`. This means that all references to `Genie.Plugins` need to be updated to use `GeniePlugins`. The `GeniePlugins` plugin is dependent on the `Genie` package and it extends the functionality of `Genie`.
### 16. `Genie.new` family of functions
All the `Genie.new` functions have been moved to `Genie.Generator` in v5.
### 17. No automatic import of `Genie` in `Main` (at REPL)
Genie v4 apps would automatically import `Genie` in `Main`, so that `Genie` would be readily available at the REPL. Starting with Genie 5, this is no longer the case and at the app's REPL it's now necessary to first run `julia> using Genie`.
### 18. Modification of `bin/server` and `bin/server.bat`
The scripts responsible for starting the app in non-interactive/serving mode need to be updated by replacing the end of the command from `s "$@"` to `-s=true "$@"`.
### 19. Update `config/initializers/logging.jl`
Update the content of the `logging.jl` initializer to this:
```julia
import Genie
Genie.Logger.initialize_logging()
```
### 20. Other
Genie 5 also changes or removes other APIs which can be generally be considered as internal. If you find other important breaking changes that have been missed, please open an issue on the Genie GitHub repository or just edit this file and submit a PR.
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 2279 | # Developing a simple API backend
Genie makes it very easy to quickly set up a REST API backend. All it takes is a few lines of code. Add these to a `rest.jl` file:
```julia
# rest.jl
using Genie
import Genie.Renderer.Json: json
Genie.config.run_as_server = true
route("/") do
(:message => "Hi there!") |> json
end
up()
```
The key bit here is `Genie.config.run_as_server = true`. This will start the server synchronously (blocking) so the `up()` function won't return (won't exit) keeping the Julia process running.
As an alternative, we can skip the `run_as_server = true` configuration and use `up(async = false)` instead.
The script can be run directly from the command line:
```shell
$ julia rest.jl
```
If you run the above code in the REPL, there is no need to set up `run_as_server = true` or `up(async = false)` because the REPL will keep the Julia process running.
## Accepting JSON payloads
One common requirement when exposing APIs is to accept `POST` payloads. That is, requests over `POST`, with a request body as a JSON encoded object. We can build an echo service like this:
```julia
using Genie, Genie.Renderer.Json, Genie.Requests
using HTTP
route("/echo", method = POST) do
message = jsonpayload()
(:echo => (message["message"] * " ") ^ message["repeat"]) |> json
end
route("/send") do
response = HTTP.request("POST", "http://localhost:8000/echo", [("Content-Type", "application/json")], """{"message":"hello", "repeat":3}""")
response.body |> String |> json
end
up(async = false)
```
Here we define two routes, `/send` and `/echo`. The `send` route makes a `HTTP` request over `POST` to `/echo`, sending a JSON payload with two values, `message` and `repeat`.
In the `/echo` route, we grab the JSON payload using the `Requests.jsonpayload()` function, extract the values from the JSON object, and output the `message` value repeated for a number of times equal to the `repeat` value.
If you run the code, the output should be
```javascript
{
echo: "hello hello hello "
}
```
If the payload contains invalid JSON, the `jsonpayload` will be set to `nothing`. You can still access the raw payload by using the `Requests.rawpayload()` function.
You can also use `rawpayload` if for example the type of request/payload is not JSON.
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 39328 | # Working with Genie apps (projects)
Working with Genie in an interactive environment can be useful – but usually we want to persist the application and
reuse it between sessions. One way to achieve this is to save it as an IJulia notebook and rerun the cells.
However, you can get the best of Genie by working with Genie apps. A Genie app is a MVC (Model-View-Controller) web
application which promotes the convention-over-configuration principle. By working with a few predefined files, within
the Genie app structure, the framework can lift a lot of weight and massively improve development productivity.
By following Genie's workflow, one instantly gets, out of the box, features like automatic module loading and reloading,
dedicated configuration files, logging, support for environments, code generators, caching, support for Genie plugins,
and much more.
In order to create a new Genie app, we need to run `Genie.Generator.newapp($app_name)`:
```julia
julia> Genie.Generator.newapp("MyGenieApp")
```
Upon executing the command, Genie will:
* make a new dir called `MyGenieApp` and `cd()` into it,
* install all the app's dependencies,
* create a new Julia project (adding the `Project.toml` and `Manifest.toml` files),
* activate the project,
* automatically load the new app's environment into the REPL,
* start the web server on the default Genie port (port 8000) and host (127.0.0.1 -- aka `localhost`).
At this point you can confirm that everything worked as expected by visiting <http://127.0.0.1:8000> in your favourite
web browser. You should see Genie's welcome page.
Next, let's add a new route. Routes are used to map request URLs to Julia functions. These functions (also called
"handler" functions) provide the response that will be sent back to the client. Routes are meant to be defined in the
dedicated `routes.jl` file (but there is no restriction enforcing this rule). Open `MyGenieApp/routes.jl` in your editor
or run the following command (making sure that you are in the app's directory):
```julia
julia> edit("routes.jl")
```
Append this at the bottom of the `routes.jl` file and save it:
```julia
# routes.jl
route("/hello") do
"Welcome to Genie!"
end
```
We are using the `route` method, passing in the "/hello" URL and an anonymous function which returns the string
"Welcome to Genie!". What this means is that for each request to the "/hello" URL, our app will invoke the route handler
function and will respond with the welcome message.
Visit <http://127.0.0.1:8000/hello> for a warm welcome!
## Working with resources
Adding our code to the `routes.jl` file works great for small projects, where you want to quickly publish features on
the web. But for larger projects we're better off using Genie's MVC structure (MVC stands for Model-View-Controller).
By employing the Model-View-Controller design pattern we can break our code into modules with clear responsibilities:
the Model is used for data access, the View renders the response to the client, and the Controller orchestrates the
interactions between Models and Views and handles requests. Modular code is easier to write, test and maintain.
A Genie app can be architected around the concept of "resources". A resource represents a business entity (something
like a user, or a product, or an account) and maps to a bundle of files (controller, model, views, etc). Resources live
under the `app/resources/` folder and each resource has its own dedicated folder, where all of its files are hosted.
For example, if we have a web app about "books", a "books" folder would be found at `app/resources/books` and will
contain all the files for publishing books on the web (usually called `BooksController.jl` for the controller,
`Books.jl` for the model, `BooksValidator.jl` for the model validator -- as well as a `views` folder for hosting all
the view files necessary for rendering books data).
---
**HEADS UP**
When creating a default Genie app, the `app/` folder might be missing. It will be automatically created the first time
you add a resource via Genie's generators.
---
## Using Controllers
Controllers are used to orchestrate interactions between client requests, Models (which handle data access), and Views
(which are responsible for rendering the responses which will be sent to the clients' web browsers). In a standard
workflow, a `route` points to a method in the controller – which is charged with building and sending the response over
the network, back to the client.
Let's add a "books" controller. Genie comes with handy generators and one of them is for creating new controllers:
### Generate the Controller
Let's generate our `BooksController`:
```julia
julia> Genie.Generator.newcontroller("Books")
[info]: New controller created at ./app/resources/books/BooksController.jl
```
Great! Let's edit `BooksController.jl` (`julia> edit("./app/resources/books/BooksController.jl")`) and add something to
it. For example, a function which returns some of Bill Gates' recommended books would be nice. Make sure that
`BooksController.jl` looks like this:
```julia
# app/resources/books/BooksController.jl
module BooksController
struct Book
title::String
author::String
end
const BillGatesBooks = Book[
Book("The Best We Could Do", "Thi Bui"),
Book("Evicted: Poverty and Profit in the American City", "Matthew Desmond"),
Book("Believe Me: A Memoir of Love, Death, and Jazz Chickens", "Eddie Izzard"),
Book("The Sympathizer", "Viet Thanh Nguyen"),
Book("Energy and Civilization, A History", "Vaclav Smil")
]
function billgatesbooks()
"
<h1>Bill Gates' list of recommended books</h1>
<ul>
$(["<li>$(book.title) by $(book.author)</li>" for book in BillGatesBooks]...)
</ul>
"
end
end
```
Our controller is just a plain Julia module where we define a `Book` struct and set up an array of book objects. We
then define a function, `billgatesbooks`, which returns an HTML string, with a `H1` heading and an unordered list of all
the books. We used an array comprehension to iterate over each book and render it in a `<li>` element. The elements of
the array are then concatenated using the splat `...` operator.
The plan is to map this function to a route and expose it on the internet.
#### Checkpoint
Before exposing it on the web, we can test the function in the REPL:
```julia
julia> using MyGenieApp.BooksController
julia> BooksController.billgatesbooks()
```
The output of the function call should be a HTML string which looks pretty much like this:
```julia
"<h1>Bill Gates' list of recommended books</h1><ul><li>The Best We Could Do by Thi Bui</li><li>Evicted:
Poverty and Profit in the American City by Matthew Desmond</li><li>Believe Me: A Memoir of Love, Death, and Jazz
Chickens by Eddie Izzard</li><li>The Sympathizer by Viet Thanh Nguyen</li><li>Energy and Civilization, A History by
Vaclav Smil</li></ul>"
```
Please make sure that it works as expected.
### Setup the route
Now, let's expose our `billgatesbooks` method on the web. We need to add a new `route` which points to it. Add these to the `routes.jl` file:
```julia
# routes.jl
using Genie
using MyGenieApp.BooksController
route("/bgbooks", BooksController.billgatesbooks)
```
In the snippet we declared that we're `using MyGenieApp.BooksController`. Do notice that Genie automatically included the
module as there was no need to explicitly include the file -- and in addition Genie will reload the source code every
time we change it. Next we defined a `route` mapping the `/bgbooks` URL and the `BooksController.billgatesbooks` function
(we say that the `BooksController.billgatesbooks` is the route handler for the `/bgbooks` URL or endpoint).
That's all! If you now visit `http://localhost:8000/bgbooks` you'll see Bill Gates' list of recommended books
(well, at least some of them, the man reads a lot!).
---
**PRO TIP**
If you would rather work with Julia instead of wrangling HTML strings, you can use Genie's `Renderer.Html` API.
It provides functions which map every standard HTML element. For instance, the `BooksController.billgatesbooks` function
can be written as follows, as an array of HTML elements:
```julia
using Genie.Renderer.Html
function billgatesbooks()
[
h1() do
"Bill Gates' list of recommended books"
end
ul() do
for_each(BillGatesBooks) do book
li() do
book.title * " by " * book.author
end
end
end
]
end
```
The `for_each` function iterates over a collection of elements and concatenates the output of each loop into the result
of the loop. We'll talk about it more soon.
---
### Adding views
However, putting HTML into the controllers is a bad idea: HTML should stay in the dedicated view files and contain as
little logic as possible. Let's refactor our code to use views instead.
The views used for rendering a resource should be placed inside the `views/` folder, within that resource's own folder
structure. So in our case, we will add an `app/resources/books/views/` folder. Just go ahead and do it, Genie does not
provide a generator for this task:
```julia
julia> mkdir(joinpath("app", "resources", "books", "views"))
```
We created the `views/` folder in `app/resources/books/`. We provided the full path as our REPL is running in the the
root folder of the app. Also, we use the `joinpath` function so that Julia creates the path in a cross-platform way.
### Naming views
Usually each controller method will have its own rendering logic – hence, its own view file. Thus, it's a good practice
to name the view files just like the methods, so that we can keep track of where they're used.
At the moment, Genie supports HTML and Markdown view files, as well as plain Julia. Their type is identified by file
extension so that's an important part. The HTML views use a `.jl.html` extension while the Markdown files go with
`.jl.md` and the Julia ones by `.jl`.
### HTML views
All right then, let's add our first view file for the `BooksController.billgatesbooks` method. Let's create an HTML
view file. With Julia:
```julia
julia> touch(joinpath("app", "resources", "books", "views", "billgatesbooks.jl.html"))
```
Genie supports a special type of dynamic HTML view, where we can embed Julia code. These are high performance compiled
views. They are _not_ parsed as strings: instead, **the HTML is converted to a Julia function that uses native Julia rendering
code and is cached to the file system and loaded like any other Julia file**. Hence, the first time you load a view, or
after you change one, you might notice a certain delay – it's the time needed to generate, compile and load the view.
On next runs (especially in production) it's going to be blazing fast!
In case you're curious, the auto-generated Julia view functions are stored by default in the `build/` folder in the app.
Feel free to take a look. The `build/` folder can be safely deleted, Genie will create it back as needed. The location
of the `build/` folder is configurable and can be changed from Genie's config.
Now all we need to do is to move the HTML code out of the controller and into the view, improving it a bit to also show
a count of the number of books. Edit the view file as follows (`julia> edit("app/resources/books/views/billgatesbooks.jl.html")`):
```html
<!-- billgatesbooks.jl.html -->
<h1>Bill Gates' top $(length(books)) recommended books</h1>
<ul>
<% for_each(books) do book %>
<li>$(book.title) by $(book.author)</li>
<% end %>
</ul>
```
As you can see, it's just plain HTML with embedded Julia. We can add Julia code by using the `<% ... %>` code block tags
– these should be used for more complex, multiline expressions. Or by using plain Julia string interpolation with `$(...)`
– for simple values outputting.
To make HTML generation more efficient, Genie provides a series of helpers, like the above `for_each` macro which allows
iterating over a collection, passing the current item into the processing function.
### Rendering views
We now need to refactor our controller to use the view, passing in the expected variables. We will use the `html` method
which renders and outputs the response as HTML. Update the definition of the `billgatesbooks` function to be as follows:
```julia
# BooksController.jl
using Genie.Renderer.Html
function billgatesbooks()
html(:books, :billgatesbooks, books = BillGatesBooks)
end
```
First, notice that we needed to add `Genie.Renderer.Html` as a dependency, to get access to the `html` method. As for
the `html` method itself, it takes as its arguments the name of the resource, the name of the view file, and a list of
keyword arguments representing view variables:
* `:books` is the name of the resource (which effectively indicates in which `views` folder Genie should look for the
view file -- in our case `app/resources/books/views`);
* `:billgatesbooks` is the name of the view file. We don't need to pass the extension, Genie will figure it out since
there's only one file with this name;
* and finally, we pass the values we want to expose in the view, as keyword arguments.
That's it – our refactored app should be ready! You can try it out for yourself at <http://localhost:8000/bgbooks>
### Markdown views
Markdown views work similar to HTML views – employing the same embedded Julia functionality. Here is how you can add a
Markdown view for our `billgatesbooks` function.
First, create the corresponding view file, using the `.jl.md` extension. Maybe with:
```julia
julia> touch(joinpath("app", "resources", "books", "views", "billgatesbooks.jl.md"))
```
Now edit the file and make sure it looks like this:
```md
<!-- app/resources/books/views/billgatesbooks.jl.md -->
# Bill Gates' $(length(books)) recommended books
$(
for_each(books) do book
"* $(book.title) by $(book.author) \n"
end
)
```
Notice that Markdown views do not support Genie's HTML embedded Julia tags `<% ... %>`. Only string interpolation
`$(...)` is accepted, but it works across multiple lines.
If you reload the page now, however, Genie will still load the HTML view. The reason is that, _if we have only one view
file_, Genie will manage. But if there's more than one, the framework won't know which one to pick. It won't error out
but will pick the default one, which is the HTML version.
It's a simple change in the `BookiesController`: we have to explicitly tell Genie which file to load, extension and all:
```julia
# BooksController.jl
function billgatesbooks()
html(:books, "billgatesbooks.jl.md", books = BillGatesBooks)
end
```
### Taking advantage of layouts
Genie's views are rendered within a layout file. Layouts are meant to render the theme of the website, or the "frame"
around the view – the elements which are common on all the pages. The layout file can include visible elements, like the
main menu or the footer. But also maybe the `<head>` tag or the assets tags (`<link>` and `<script>` tags for loading
CSS and JavaScript files in all the pages).
Every Genie app has a main layout file which is used by default – it can be found in `app/layouts/` and is called `app.jl.html`. It looks like this:
```html
<!-- app/layouts/app.jl.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<title>Genie :: The highly productive Julia web framework</title>
<!-- link rel="stylesheet" href="/css/application.css" / -->
</head>
<body>
<%
@yield
%>
<!-- script src="/js/application.js"></script -->
</body>
</html>
```
We can edit it. For example, add this right under the opening `<body>` tag, just above the `<%` tag:
```html
<h1>Welcome to top books</h1>
```
If you reload the page at <http://localhost:8000/bgbooks> you will see the new heading.
But we don't have to stick to the default; we can add additional layouts. Let's suppose that we have, for example, an
admin area which should have a completely different theme. We can add a dedicated layout for that:
```julia
julia> touch(joinpath("app", "layouts", "admin.jl.html"))
```
Now edit it (`julia> edit("app/layouts/admin.jl.html")`) and make it look like this:
```html
<!-- app/layouts/admin.jl.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<title>Genie Admin</title>
</head>
<body>
<h1>Books admin</h1>
<%
@yield
%>
</body>
</html>
```
If we want to apply it, we must instruct our `BooksController` to use it. The `html` function takes a keyword argument
named `layout`, for the layout file. Update the `billgatesbooks` function to look like this:
```julia
# BooksController.jl
function billgatesbooks()
html(:books, :billgatesbooks, books = BillGatesBooks, layout = :admin)
end
```
Reload the page and you'll see the new heading.
#### The `@yield` instruction
There is a special instruction in the layouts: `@yield`. It outputs the contents of the view as rendered through the
controller. So where this macro is present, Genie will output the HTML resulting from rendering the view by executing
the route handler function within the controller.
#### Using view paths
For very simple applications the MVC and the resource-centric approaches might involve too much boilerplate. In such
cases, we can simplify the code by referencing the view (and layout) by file path, ex:
```julia
# BooksController.jl
using Genie.Renderer
function billgatesbooks()
html(path"app/resources/books/views/billgatesbooks.jl.html", books = BillGatesBooks, layout = path"app/layouts/app.jl.html")
end
```
### Rendering JSON views
A common use case for web apps is to serve as backends for RESTful APIs. For such cases, JSON is the preferred data
format. You'll be happy to hear that Genie has built-in support for JSON responses. Let's add an endpoint for our API –
which will render Bill Gates' books as JSON.
We can start in the `routes.jl` file, by appending this
```julia
route("/api/v1/bgbooks", BooksController.API.billgatesbooks)
```
Next, in `BooksController.jl`, append the extra logic at the end of the file, before the closing `end`. The whole file should look like this:
```julia
# BooksController.jl
module BooksController
using Genie.Renderer.Html
struct Book
title::String
author::String
end
const BillGatesBooks = Book[
Book("The Best We Could Do", "Thi Bui"),
Book("Evicted: Poverty and Profit in the American City", "Matthew Desmond"),
Book("Believe Me: A Memoir of Love, Death, and Jazz Chickens", "Eddie Izzard"),
Book("The Sympathizer!", "Viet Thanh Nguyen"),
Book("Energy and Civilization, A History", "Vaclav Smil")
]
function billgatesbooks()
html(:books, :billgatesbooks, layout = :admin, books = BillGatesBooks)
end
module API
using ..BooksController
using Genie.Renderer.Json
function billgatesbooks()
json(BooksController.BillGatesBooks)
end
end
end
```
We nested an API module within the `BooksController` module, where we defined another `billgatesbooks` function which outputs a JSON.
If you go to `http://localhost:8000/api/v1/bgbooks` it should already work as expected.
#### JSON views
However, we have just committed one of the cardinal sins of API development. We have just forever coupled our internal
data structure to its external representation. This will make future refactoring very complicated and error prone as any
changes in the data will break the client's integrations. The solution is to, again, use views, to fully control how we
render our data – and decouple the data structure from its rendering on the web.
Genie has support for JSON views – these are plain Julia files which have the ".json.jl" extension.
Let's add one in our `views/` folder:
```julia
julia> touch(joinpath("app", "resources", "books", "views", "billgatesbooks.json.jl"))
```
We can now create a proper response. Put this in the view file:
```julia
# app/resources/books/views/billgatesbooks.json.jl
"Bill Gates' list of recommended books" => books
```
Final step, instructing `BooksController` to render the view. Simply replace the existing `billgatesbooks` function
within the `API` sub-module with the following:
```julia
function billgatesbooks()
json(:books, :billgatesbooks, books = BooksController.BillGatesBooks)
end
```
This should hold no surprises – the `json` function is similar to the `html` one we've seen before. So now we're
rendering a custom JSON response. That's all – everything should work!
---
**HEADS UP**
#### Why JSON views have the extension ending in `.jl` but HTML and Markdown views do not?
Good question! The extension of the views is chosen in order to preserve correct syntax highlighting in the IDE/code editor.
Since practically HTML and Markdown views are HTML and Markdown files with some embedded Julia code, we want to use the
HTML or Markdown syntax highlighting. For JSON views, we use pure Julia, so we want Julia syntax highlighting.
---
## Accessing databases with `SearchLight` models
You can get the most out of Genie by pairing it with its seamless ORM layer, SearchLight. SearchLight, a native Julia
ORM, provides excellent support for working with relational databases. The Genie + SearchLight combo can be used to
productively develop CRUD (Create-Read-Update-Delete) apps.
---
**HEADS UP**
CRUD stands for Create-Read-Update-Delete and describes the data workflow in many web apps, where resources are created,
read (listed), updated, and deleted.
---
SearchLight represents the "M" part in Genie's MVC architecture (thus, the Model layer).
Let's begin by adding SearchLight to our Genie app. All Genie apps manage their dependencies in their own Julia environment,
through their `Project.toml` and `Manifest.toml` files.
So we need to make sure that we're in `pkg> ` mode first (which is entered by typing `]` in julian mode, ie: `julia>]`).
The cursor should change to `(MyGenieApp) pkg>`.
Next, we add `SearchLight`:
```julia
(MyGenieApp) pkg> add SearchLight
```
### Adding a database adapter
`SearchLight` provides a database agnostic API for working with various backends (at the moment, MySQL, SQLite, Postgres
and Oracle). Thus, we also need to add the specific adapter. To keep things simple, let's use SQLite for our app.
Hence, we'll need the `SearchLightSQLite` package:
```julia
(MyGenieApp) pkg> add SearchLightSQLite
```
### Setup the database connection
Genie is designed to seamlessly integrate with SearchLight and provides access to various database oriented generators.
First we need to tell Genie/SearchLight how to connect to the database. Let's use them to set up our database support.
Run this in the Genie/Julia REPL:
```julia
julia> Genie.Generator.db_support()
```
The command will add a `db/` folder within the root of the app. What we're looking for is the `db/connection.yml` file
which tells SearchLight how to connect to the database. Let's edit it. Make the file look like this:
```yaml
env: ENV["GENIE_ENV"]
dev:
adapter: SQLite
database: db/books.sqlite
config:
```
This instructs SearchLight to run in the environment of the current Genie app (by default `dev`), using `SQLite` for
the adapter (backend) and a database stored at `db/books.sqlite` (the database will be created automatically if it does
not exist). We could pass extra configuration options in the `config` object, but for now we don't need anything else.
---
**HEADS UP**
If you are using a different adapter, make sure that the database configured already exists and that the configured user
can successfully access it -- SearchLight will not attempt to create the database.
---
Now we can ask SearchLight to load it up:
```julia
julia> using SearchLight
julia> SearchLight.Configuration.load()
Dict{String,Any} with 4 entries:
"options" => Dict{String,String}()
"config" => nothing
"database" => "db/books.sqlite"
"adapter" => "SQLite"
```
Let's just go ahead and try it out by connecting to the DB:
```julia
julia> using SearchLightSQLite
julia> SearchLight.Configuration.load() |> SearchLight.connect
```
This should return a `SQLite.DB("db/books.sqlite")` object. If that is the case, the connection succeeded and we got
back a SQLite database handle.
---
**PRO TIP**
Each database adapter exposes a `CONNECTIONS` collection where we can access the connection:
```julia
julia> SearchLightSQLite.CONNECTIONS
1-element Array{SQLite.DB,1}:
SQLite.DB("db/books.sqlite")
```
---
Awesome! If all went well you should have a `books.sqlite` database in the `db/` folder.
```julia
shell> tree db
db
├── books.sqlite
├── connection.yml
├── migrations
└── seeds
```
### Managing the database schema with `SearchLight` migrations
Database migrations provide a way to reliably, consistently and repeatedly apply (and undo) changes to the structure of
your database (known as "schema transformations"). They are specialised scripts for adding, removing and altering DB
tables – these scripts are placed under version control and are managed by a dedicated system which knows which scripts
have been run and which not, and is able to run them in the correct order.
SearchLight needs its own DB table to keep track of the state of the migrations so let's set it up:
```julia
julia> SearchLight.Migrations.init()
[ Info: Created table schema_migrations
```
This command sets up our database with the needed table in order to manage migrations.
---
**PRO TIP**
You can use the SearchLight API to execute random queries against the database backend. For example we can confirm that
the table is really there:
```julia
julia> SearchLight.query("SELECT name FROM sqlite_master WHERE type ='table' AND name NOT LIKE 'sqlite_%'")
┌ Info: SELECT name FROM sqlite_master WHERE type ='table' AND name NOT LIKE 'sqlite_%'
└
1×1 DataFrames.DataFrame
│ Row │ name │
│ │ String⍰ │
├─────┼───────────────────┤
│ 1 │ schema_migrations │
```
The result is a `DataFrame` object.
---
### Creating our Book model
SearchLight, just like Genie, uses the convention-over-configuration design pattern. It prefers for things to be setup
in a certain way and provides sensible defaults, versus having to define everything in extensive configuration files.
And fortunately, we don't even have to remember what these conventions are, as SearchLight also comes with an extensive
set of generators.
Lets ask SearchLight to create a new model:
```julia
julia> SearchLight.Generator.newresource("Book")
[ Info: New model created at /Users/adrian/Dropbox/Projects/MyGenieApp/app/resources/books/Books.jl
[ Info: New table migration created at /Users/adrian/Dropbox/Projects/MyGenieApp/db/migrations/2020020909574048_create_table_books.jl
[ Info: New validator created at /Users/adrian/Dropbox/Projects/MyGenieApp/app/resources/books/BooksValidator.jl
[ Info: New unit test created at /Users/adrian/Dropbox/Projects/MyGenieApp/test/books_test.jl
```
SearchLight has created the `Books.jl` model, the `*_create_table_books.jl` migration file, the `BooksValidator.jl` model
validator and the `books_test.jl` test file.
---
**HEADS UP**
The first part of the migration file will be different for you!
The `*_create_table_books.jl` file will be named differently as the first part of the name is the file creation timestamp.
This timestamp part guarantees that names are unique and file name clashes are avoided (for example when working as a
team a creating similar migration files).
---
#### Writing the table migration
Lets begin by writing the migration to create our books table. SearchLight provides a powerful DSL for writing migrations.
Each migration file needs to define two methods: `up` which applies the changes – and `down` which undoes the effects of
the `up` method. So in our `up` method we want to create the table – and in `down` we want to drop the table.
The naming convention for tables in SearchLight is that the table name should be pluralized (`books`) – because a table
contains multiple _books_ (and each row represents an object, a single _book_).
But don't worry, the migration file should already be pre-populated with the correct table name.
Edit the `db/migrations/*_create_table_books.jl` file and make it look like this:
```julia
module CreateTableBooks
import SearchLight.Migrations: create_table, column, primary_key, add_index, drop_table
function up()
create_table(:books) do
[
primary_key()
column(:title, :string, limit = 100)
column(:author, :string, limit = 100)
]
end
add_index(:books, :title)
add_index(:books, :author)
end
function down()
drop_table(:books)
end
end
```
The code is pretty easy to follow: in the `up` function we call `create_table` and pass an array of columns: a primary
key, a `title` column and an `author` column (both strings have a max length of 100). We also add two indices (one on
the `title` and the other on the `author` columns). As for the `down` method, it invokes the `drop_table` function to
remove the table.
#### Running the migration
We can see what SearchLight knows about our migrations with the `SearchLight.Migrations.status` command:
```julia
julia> SearchLight.Migrations.status()
| | Module name & status |
| | File name |
|---|----------------------------------------|
| | CreateTableBooks: DOWN |
| 1 | 2020020909574048_create_table_books.jl |
```
So our migration is in the `down` state – meaning that its `up` method has not been run. We can easily fix this:
```julia
julia> SearchLight.Migrations.last_up()
[ Info: Executed migration CreateTableBooks up
```
If we recheck the status, the migration is up:
```julia
julia> SearchLight.Migrations.status()
| | Module name & status |
| | File name |
|---|----------------------------------------|
| | CreateTableBooks: UP |
| 1 | 2020020909574048_create_table_books.jl |
```
Our table is ready!
#### Defining the model
Now it's time to edit our model file at `app/resources/books/Books.jl`. Another convention in SearchLight is that we're
using the pluralized name (`Books`) for the module – because it's for managing multiple books. And within it we define a
type (a `mutable struct`), called `Book` – which represents an item (a single book) which maps to a row in the underlying database.
Edit the `Books.jl` file to make it look like this:
```julia
# Books.jl
module Books
import SearchLight: AbstractModel, DbId, save!
import Base: @kwdef
export Book
@kwdef mutable struct Book <: AbstractModel
id::DbId = DbId()
title::String = ""
author::String = ""
end
end
```
We defined a `mutable struct` which matches our previous `Book` type by using the `@kwdef` macro, in order to also define
a keyword constructor, as SearchLight needs it.
#### Using our model
To make things more interesting, we should import our current books into the database. Add this function to the
`Books.jl` module, under the `Book()` constructor definition (just above the module's closing `end`):
```julia
# Books.jl
function seed()
BillGatesBooks = [
("The Best We Could Do", "Thi Bui"),
("Evicted: Poverty and Profit in the American City", "Matthew Desmond"),
("Believe Me: A Memoir of Love, Death, and Jazz Chickens", "Eddie Izzard"),
("The Sympathizer!", "Viet Thanh Nguyen"),
("Energy and Civilization, A History", "Vaclav Smil")
]
for b in BillGatesBooks
Book(title = b[1], author = b[2]) |> save!
end
end
```
#### Auto-loading the DB configuration
Now, to try things out. Genie takes care of loading all our resource files for us when we load the app. To do this,
Genie comes with a special file called an initializer, which automatically loads the database configuration and sets up
SearchLight. Check `config/initializers/searchlight.jl` to see how this is done.
---
**Heads up!**
All the `*.jl` files placed into the `config/initializers/` folder are automatically included by Genie upon starting the
Genie app. They are included early (upon initialisation), before the controllers, models, views, are loaded.
---
#### Trying it out
Now it's time to restart our REPL session and test our app. Close the Julia REPL session to exit to the OS command line and run:
```bash
$ bin/repl
```
On Windows you will need to run `bin/repl.bat` instead.
The `repl` executable script placed within the app's `bin/` folder starts a new Julia REPL session and loads the
applications' environment. Everything should be automatically loaded now, DB configuration included - so we can invoke
the previously defined `seed` function to insert the books:
```julia
julia> using MyGenieApp.Books
julia> Books.seed()
```
There should be a list of queries showing how the data is inserted in the DB:
```julia
julia> Books.seed()
[ Info: INSERT INTO books ("title", "author") VALUES ('The Best We Could Do', 'Thi Bui')
[ Info: INSERT INTO books ("title", "author") VALUES ('Evicted: Poverty and Profit in the American City', 'Matthew Desmond')
# output truncated
```
If you want to make sure all went right (although trust me, it did, otherwise SearchLight would've thrown an `Exception`!),
just ask SearchLight to retrieve the books we inserted:
```julia
julia> using SearchLight
julia> all(Book)
[ Info: 2020-02-09 13:29:32 SELECT "books"."id" AS "books_id", "books"."title" AS "books_title", "books"."author" AS "books_author" FROM "books" ORDER BY books.id ASC
5-element Array{Book,1}:
Book
| KEY | VALUE |
|----------------|----------------------|
| author::String | Thi Bui |
| id::DbId | 1 |
| title::String | The Best We Could Do |
Book
| KEY | VALUE |
|----------------|--------------------------------------------------|
| author::String | Matthew Desmond |
| id::DbId | 2 |
| title::String | Evicted: Poverty and Profit in the American City |
# output truncated
```
The `SearchLight.all` method returns all the `Book` items from the database.
All good!
The next thing we need to do is to update our controller to use the model.
Make sure that `app/resources/books/BooksController.jl` reads like this:
```julia
# BooksController.jl
module BooksController
using Genie.Renderer.Html
using SearchLight
using MyGenieApp.Books
function billgatesbooks()
html(:books, :billgatesbooks, books = all(Book))
end
module API
using ..BooksController
using Genie.Renderer.Json
using SearchLight
using MyGenieApp.Books
function billgatesbooks()
json(:books, :billgatesbooks, books = all(Book))
end
end
end
```
Our JSON view needs a bit of tweaking too:
```julia
# app/resources/books/views/billgatesbooks.json.jl
"Bill's Gates list of recommended books" => [Dict("author" => b.author, "title" => b.title) for b in books]
```
Now if we just start the server we'll be able to see the list of books served from the database:
```julia
# Start the server
julia> up()
```
The `up` method starts up the web server and takes us back to the interactive Julia REPL prompt.
Now, if, for example, we navigate to <http://localhost:8000/api/v1/bgbooks>, the output should match the following JSON document:
```json
{
"Bill's Gates list of recommended books": [
{
"author": "Thi Bui",
"title": "The Best We Could Do"
},
{
"author": "Matthew Desmond",
"title": "Evicted: Poverty and Profit in the American City"
},
{
"author": "Eddie Izzard",
"title": "Believe Me: A Memoir of Love, Death, and Jazz Chickens"
},
{
"author": "Viet Thanh Nguyen",
"title": "The Sympathizer!"
},
{
"author": "Vaclav Smil",
"title": "Energy and Civilization, A History"
}
]
}
```
Let's add a new book to see how it works. We'll create a new `Book` item and persist it using the `SearchLight.save!` method:
```julia
julia> newbook = Book(title = "Leonardo da Vinci", author = "Walter Isaacson")
Book
| KEY | VALUE |
|----------------|-------------------|
| author::String | Walter Isaacson |
| id::DbId | NULL |
| title::String | Leonardo da Vinci |
julia> save!(newbook)
[ Info: INSERT INTO books ("title", "author") VALUES ('Leonardo da Vinci', 'Walter Isaacson')
[ Info: ; SELECT CASE WHEN last_insert_rowid() = 0 THEN -1 ELSE last_insert_rowid() END AS id
[ Info: SELECT "books"."id" AS "books_id", "books"."title" AS "books_title", "books"."author" AS "books_author" FROM "books" WHERE "id" = 6 ORDER BY books.id ASC
Book
| KEY | VALUE |
|----------------|-------------------|
| author::String | Walter Isaacson |
| id::DbId | 6 |
| title::String | Leonardo da Vinci |
```
Calling the `save!` method, SearchLight has persisted the object in the database and then retrieved it and returned it
(notice the updated `id::DbId` field).
The same `save!` operation can be written as a one-liner:
```julia
julia> Book(title = "Leonardo da Vinci", author = "Walter Isaacson") |> save!
```
---
**HEADS UP**
If you also run the one-liner `save!` example, it will add the same book again.
No problem, but if you want to remove it, you can use the `delete` method:
```julia
julia> duplicated_book = find(Book, title = "Leonardo da Vinci")[end]
julia> delete(duplicated_bookd)
[ Info: DELETE FROM books WHERE id = '7'
Book
| KEY | VALUE |
|----------------|-------------------|
| author::String | Walter Isaacson |
| id::DbId | NULL |
| title::String | Leonardo da Vinci |
```
---
If you reload the page at <http://localhost:8000/bgbooks> the new book should show up.
```json
{
"Bill's Gates list of recommended books": [
{
"author": "Thi Bui",
"title": "The Best We Could Do"
},
{
"author": "Matthew Desmond",
"title": "Evicted: Poverty and Profit in the American City"
},
{
"author": "Eddie Izzard",
"title": "Believe Me: A Memoir of Love, Death, and Jazz Chickens"
},
{
"author": "Viet Thanh Nguyen",
"title": "The Sympathizer!"
},
{
"author": "Vaclav Smil",
"title": "Energy and Civilization, A History"
},
{
"author": "Walter Isaacson",
"title": "Leonardo da Vinci"
}
]
}
```
---
**PRO TIP**
SearchLight exposes two similar data persistence methods: `save!` and `save`. They both perform the same action
(persisting the object to the database), but `save` will return a `Bool` of value `true` to indicate that the operation
was successful or a `Bool` of value `false` to indicate that the operation has failed. While the `save!` variant will
return the persisted object upon success or will throw an exception on failure.
---
## Congratulations
You have successfully finished the first part of the step by step walkthrough - you have mastered the Genie basics,
allowing you to set up a new app, register routes, add resources (controllers, models, and views), add database support,
version the database schema with migrations, and execute basic queries with SearchLight!
In the next part we'll look at more advanced topics like handling forms and file uploads, templates rendering, interactivity and more.
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 14685 | # Working With Genie Apps: Intermediate Topics
**WARNING: THIS PAGE IS UNDER CONSTRUCTION -- THE CONTENT IS USABLE BUT INCOMPLETE**
## Handling forms
Now, the problem is that Bill Gates reads – a lot! It would be much easier if we would allow our users to add a few books themselves, to give us a hand.
But since, obviously, we're not going to give them access to our Julia REPL, we should setup a web page with a form. Let's do it.
We'll start by adding the new routes:
```julia
# routes.jl
route("/bgbooks/new", BooksController.new)
route("/bgbooks/create", BooksController.create, method = POST, named = :create_book)
```
The first route will be used to display the page with the new book form. The second will be the target page for
submitting our form - this page will accept the form's payload. Please note that it's configured to match `POST` requests
and that we gave it a name. We'll use the name in our form so that Genie will dynamically generate the correct links to
the corresponding URL (to avoid hard coding URLs).
This way we'll make sure that our form will always submit to the right URL, even if we change the route (as long as we
don't change the name).
Now, to add the methods in `BooksController`. Add these definition under the `billgatesbooks` function (make sure you
add them in `BooksController`, not in `BooksController.API`):
```julia
# BooksController.jl
function new()
html(:books, :new)
end
function create()
# code here
end
```
The `new` method should be clear: we'll just render a view file called `new`. As for `create`, for now it's just a placeholder.
Next, to add our view. Add a blank file called `new.jl.html` in `app/resources/books/views`. Using Julia:
```julia
julia> touch("app/resources/books/views/new.jl.html")
```
Make sure that it has this content:
```html
<!-- app/resources/books/views/new.jl.html -->
<h2>Add a new book recommended by Bill Gates</h2>
<p>
For inspiration you can visit <a href="https://www.gatesnotes.com/Books" target="_blank">Bill Gates' website</a>
</p>
<form action="$(Genie.Router.linkto(:create_book))" method="POST">
<input type="text" name="book_title" placeholder="Book title" /><br />
<input type="text" name="book_author" placeholder="Book author" /><br />
<input type="submit" value="Add book" />
</form>
```
Notice that the form's action calls the `linkto` method, passing in the name of the route to generate the URL, resulting
in the following HTML: `<form method="POST" action="/bgbooks/create">`.
We should also update the `BooksController.create` method to do something useful with the form data. Let's make it
create a new book, persist it to the database and redirect to the list of books. Here is the code:
```julia
# BooksController.jl
using Genie.Router, Genie.Renderer
function create()
Book(title = params(:book_title), author = params(:book_author)) |> save && redirect(:get_bgbooks)
end
```
A few things are worth pointing out in this snippet:
* again, we're accessing the `params` collection to extract the request data, in this case passing in the names of our
form's inputs as parameters.
We need to bring `Genie.Router` into scope in order to access `params`;
* we're using the `redirect` method to perform a HTTP redirect. As the argument we're passing in the name of the route,
just like we did with the form's action. However, we didn't set any route to use this name. It turns out that Genie
gives default names to all the routes.
We can use these – but a word of notice: **these names are generated using the properties of the route, so if the route
changes it's possible that the name will change too**.
So either make sure your route stays unchanged – or explicitly name your routes. The autogenerated name, `get_bgbooks`
corresponds to the method (`GET`) and the route (`bgbooks`).
In order to get info about the defined routes you can use the `Router.named_routes` function:
```julia
julia> Router.named_routes()
OrderedCollections.OrderedDict{Symbol, Genie.Router.Route} with 6 entries:
:get_bgbooks => [GET] /bgbooks => billgatesbooks | :get_bgbooks
:get_bgbooks_new => [GET] /bgbooks/new => new | :get_bgbooks_new
:get => [GET] / => () | :get
:get_api_v1_bgbooks => [GET] /api/v1/bgbooks | :get_api_v1_bgbooks
:create_book => [POST] /bgbooks/create | :create_book
```
Let's try it out. Input something and submit the form. If everything goes well a new book will be persisted to the
database – and it will be added at the bottom of the list of books.
---
## Uploading files
Our app looks great -- but the list of books would be so much better if we'd display the covers as well. Let's do it!
### Modify the database
The first thing we need to do is to modify our table to add a new column, for storing a reference to the name of the cover image.
Per best practices, we'll use database migrations to modify the structure of our table:
```julia
julia> SearchLight.Generator.newmigration("add cover column")
[debug] New table migration created at db/migrations/2019030813344258_add_cover_column.jl
```
Now we need to edit the migration file - please make it look like this:
```julia
# db/migrations/*_add_cover_column.jl
module AddCoverColumn
import SearchLight.Migrations: add_column, add_index
# SQLite does not support column removal so the `remove_column` method is not implemented in the SearchLightSQLite adapter
# If using SQLite leave the next line commented -- otherwise uncomment it
# import SearchLight.Migrations: remove_column
function up()
add_column(:books, :cover, :string)
end
function down()
# if using the SQLite backend, leave the next line commented -- otherwise uncomment it
# remove_column(:books, :cover)
end
end
```
Looking good - lets ask SearchLight to run it:
```julia
julia> SearchLight.Migration.lastup()
[debug] Executed migration AddCoverColumn up
```
If you want to double check, ask SearchLight for the migrations status:
```julia
julia> SearchLight.Migration.status()
| | Module name & status |
| | File name |
|---|----------------------------------------|
| | CreateTableBooks: UP |
| 1 | 2018100120160530_create_table_books.jl |
| | AddCoverColumn: UP |
| 2 | 2019030813344258_add_cover_column.jl |
```
Perfect! Now we need to add the new column as a field to the `Books.Book` model:
```julia
module Books
using SearchLight, SearchLight.Validation, BooksValidator
export Book
Base.@kwdef mutable struct Book <: AbstractModel
id::DbId = DbId()
title::String = ""
author::String = ""
cover::String = ""
end
end
```
As a quick test we can extend our JSON view and see that all goes well - make it look like this:
```julia
# app/resources/books/views/billgatesbooks.json.jl
"Bill's Gates list of recommended books" => [Dict("author" => b.author,
"title" => b.title,
"cover" => b.cover) for b in books]
```
If we navigate <http://localhost:8000/api/v1/bgbooks> you should see the newly added "cover" property (empty, but present).
##### Heads up!
Sometimes Julia/Genie/Revise fails to update `structs` on changes. If you get an error saying that `Book` does not have
a `cover` field or that it can not be changed, you'll need to restart the Genie app.
### File uploading
Next step, extending our form to upload images (book covers). Please edit the `new.jl.html` view file as follows:
```html
<h3>Add a new book recommended by Bill Gates</h3>
<p>
For inspiration you can visit <a href="https://www.gatesnotes.com/Books" target="_blank">Bill Gates' website</a>
</p>
<form action="$(Genie.Router.linkto(:create_book))" method="POST" enctype="multipart/form-data">
<input type="text" name="book_title" placeholder="Book title" /><br />
<input type="text" name="book_author" placeholder="Book author" /><br />
<input type="file" name="book_cover" /><br />
<input type="submit" value="Add book" />
</form>
```
The new bits are:
* we added a new attribute to our `<form>` tag: `enctype="multipart/form-data"`. This is required in order to support files payloads.
* there's a new input of type file: `<input type="file" name="book_cover" />`
You can see the updated form by visiting <http://localhost:8000/bgbooks/new>
Now, time to add a new book, with the cover! How about "Identity" by Francis Fukuyama? Sounds good.
You can use whatever image you want for the cover, or maybe borrow the one from Bill Gates, I hope he won't mind
<https://www.gatesnotes.com/-/media/Images/GoodReadsBookCovers/Identity.png>.
Just download the file to your computer so you can upload it through our form.
Almost there - now to add the logic for handling the uploaded file server side. Please update the
`BooksController.create` method to look like this:
```julia
# BooksController
function create()
cover_path = if haskey(filespayload(), "book_cover")
path = joinpath("img", "covers", filespayload("book_cover").name)
write(joinpath("public", path), IOBuffer(filespayload("book_cover").data))
path
else
""
end
Book( title = params(:book_title),
author = params(:book_author),
cover = cover_path) |> save && redirect(:get_bgbooks)
end
```
Also, very important, you need to make sure that `BooksController` is `using Genie.Requests`.
Regarding the code, there's nothing very fancy about it. First we check if the files payload contains an entry for our `book_cover` input.
If yes, we compute the path where we want to store the file, write the file, and store the path in the database.
**Please make sure that you create the folder `covers/` within `public/img/`**.
Great, now let's display the images. Let's start with the HTML view - please edit
`app/resources/books/views/billgatesbooks.jl.html` and make sure it has the following content:
```html
<!-- app/resources/books/views/billgatesbooks.jl.html -->
<h1>Bill's Gates top $( length(books) ) recommended books</h1>
<ul>
<%
for_each(books) do book
%>
<li><img src='$( isempty(book.cover) ? "img/docs.png" : book.cover )' width="100px" /> $(book.title) by $(book.author)</li>
<%
end
%>
</ul>
```
Here we check if the `cover` property is not empty, and display the actual cover. Otherwise we show a placeholder image.
You can check the result at <http://localhost:8000/bgbooks>
As for the JSON view, it already does what we want - you can check that the `cover` property is now outputted, as stored
in the database: <http://localhost:8000/api/v1/bgbooks>
Success, we're done here!
#### Heads up!
In production you will have to make the upload code more robust - the big problem here is that we store the cover file
as it comes from the user which can lead to name clashes and files being overwritten - not to mention security vulnerabilities.
A more robust way would be to compute a hash based on author and title and rename the cover to that.
### One more thing
So far so good, but what if we want to update the books we have already uploaded? It would be nice to add those missing covers.
We need to add a bit of functionality to include editing features.
First things first - let's add the routes. Please add these two new route definitions to the `routes.jl` file:
```julia
route("/bgbooks/:id::Int/edit", BooksController.edit)
route("/bgbooks/:id::Int/update", BooksController.update, method = POST, named = :update_book)
```
We defined two new routes. The first will display the book object in the form, for editing. While the second will take
care of actually updating the database, server side. For both routes we need to pass the id of the book that we want to
edit - and we want to constrain it to an `Int`. We express this as the `/:id::Int/` part of the route.
We also want to:
* reuse the form which we have defined in `app/resources/books/views/new.jl.html`
* make the form aware of whether it's used to create a new book, or for editing an existing one respond accordingly by setting the correct `action`
* pre-fill the inputs with the book's info when editing a book.
OK, that's quite a list and this is where things become interesting. This is an important design pattern for CRUD web apps.
In order to simplify the rendering of the form, we will always pass a book object into it.
When editing a book it will be the book corresponding to the `id` passed into the `route`. And when creating a new book,
it will be just an empty book object we'll create and then dispose of.
#### Using view partials
First, let's set up the views. In `app/resources/books/views/` please create a new file called `form.jl.html`.
Then, from `app/resources/books/views/new.jl.html` cut the `<form>` code. That is, everything between the opening and closing `<form>...</form>` tags.
Paste it into the newly created `form.jl.html` file. Now, back to `new.jl.html`, instead of the previous `<form>...</form>` code add:
```julia
<% partial("app/resources/books/views/form.jl.html", context = @__MODULE__) %>
```
This line, as the `partial` function suggests, includes a view partial, which is a part of a view file, effectively
including a view within another view. Notice that we're explicitly passing the `context` so Genie can set the correct
variable scope when including the partial.
You can reload the `new` page to make sure that everything still works: <http://localhost:8000/bgbooks/new>
Now, let's add an Edit option to our list of books. Please go back to our list view file, `billgatesbooks.jl.html`.
Here, for each iteration, within the `for_each` block we'll want to dynamically link to the edit page for the corresponding book.
##### `for_each` with view partials
However, this `for_each` which renders a Julia string is very ugly - and we now know how to refactor it, by using a view partial.
Let's do it. First, replace the body of the `for_each` block:
```html
<!-- app/resources/books/views/billgatesbooks.jl.html -->
"""<li><img src='$( isempty(book.cover) ? "img/docs.png" : book.cover )' width="100px" /> $(book.title) by $(book.author)"""
```
with:
```julia
partial("app/resources/books/views/book.jl.html", book = book, context = @__MODULE__)
```
Notice that we are using the `partial` function and we pass the book object into our view, under the name `book`
(will be accessible in `book` inside the view partial). Again, we're passing the scope's `context` (our controller object).
Next, create the `book.jl.html` in `app/resources/books/views/`, for example with
```julia
julia> touch("app/resources/books/views/book.jl.html")
```
Add this content to it:
TO BE CONTINUED
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 1577 | # Welcome to Genie
## The Highly Productive Web Framework for Julia
Genie is a full stack web framework for the Julia programming language. Genie's goals are: excellent developer
productivity, great run-time performance, and best practices and security by default.
The Genie web framework follows in the footsteps of mainstream full stack web frameworks like Ruby on Rails and Django,
while staying 100% true to its Julia roots. Genie's architecture and development is inspired by the best features present in other
frameworks, but not by their design. Genie takes a no-magic no-nonsense approach by doing things the Julia way:
`Controllers` are plain Julia modules, `Models` leverage types and multiple dispatch, Genie apps are nothing but Julia
projects, versioning and dependency management is provided by Julia's own `Pkg`, and code loading and reloading is automatically
set up with `Revise`.
Genie also takes inspiration from Julia's "start simple, grow as needed" philosophy, by allowing developers to bootstrap
an app in the REPL or in a notebook, or easily create web services and APIs with just a few lines of code.
As the projects grow more complex, Genie allows adding progressively more structure, by exposing a micro-framework
which offers features like powerful routing, flexible logging, support for environments, view templates, etc.
If database persistence is needed, support for Genie's ORM, SearchLight, can be added at any time. Finally, the full MVC
structure can be used in order to develop and maintain more complex, end-to-end, web applications.
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 3423 | # Loading and starting Genie apps
Genie apps are Julia projects that are composed of multiple modules distributed over multiple files.
Loading a Genie app will bring into scope all the app's files, including the main app module, controllers, models, etcetera.
## Starting a Genie REPL session MacOS / Linux
The quickest way to load an existing app in an interactive REPL is by executing `bin/repl` in the os shell,
while in the app's root folder.
```sh
$ bin/repl
```
The Genie app will be loaded.
In order to start the web server, you can next execute:
```julia
julia> up()
```
If you want to directly start the server without having access to the interactive REPL, use `bin/server` instead of `bin/repl`:
```sh
$ bin/server
```
This will automatically start the web server _in non interactive mode_.
## Starting a Genie REPL on Windows
On Windows the workflow is similar to macOS and Linux, but dedicated Windows scripts, `repl.bat`, `server.bat` are
provided inside the project folder, within the `bin/` directory. Double click them or execute them in the os shell
(cmd, Windows Terminal, PowerShell, etc) to start an interactive REPL session or a server session, respectively,
as explained in the previous paragraphs (the *nix and the Windows scripts run int the same way).
---
**HEADS UP**
It is possible that the scripts in the `bin/` folder are missing - this is usually the case if the
app was generated on an operating system (ex *nix) and ported to a different one (ex Windows).
You can create them at anytime by running the generator in the Genie/Julia REPL (at the root of the Genie project).
To generate the Windows scripts:
```julia
julia> using Genie
julia> Genie.Generator.setup_windows_bin_files()
```
And for the *nix scripts:
```julia
julia> Genie.Generator.setup_nix_bin_files()
```
Alternatively, we can pass the path where we want the files to be created as the argument to `setup_*_bin_files`:
```julia
julia> Genie.Generator.setup_windows_bin_files("path/to/your/Genie/project")
```
## REPL / Jupyter / Pluto / VSCode / other Julia environment
You might need to make the local package environment available, if it's not already activated:
```julia
using Pkg
Pkg.activate(".")
```
Then:
```julia
using Genie
Genie.loadapp()
```
This will assume that we're already in the app's root folder, and load the app (in other words that the `bootstrap.jl` file
is in the current working directory). Otherwise you can also pass the path to the Genie app's folder as the argument for
`loadapp`.
```julia
julia> Genie.loadapp("path/to/your/Genie/project")
```
## Manual loading in Julia's REPL
In order to load a Genie app within an open Julia REPL session, first make sure that you're in the root dir of a Genie app.
This is the project's folder and you can tell by the fact that there should be a `bootstrap.jl` file, plus Julia's
`Project.toml` and `Manifest.toml` files, amongst others. You can `julia> cd(...)` or `shell> cd ...` your way into the
folder of the Genie app.
Next, from within the active Julia REPL session, we have to activate the local package environment:
```julia
julia> ] # enter pkg> mode
pkg> activate .
```
Then, back to the julian prompt, run the following to load the Genie app:
```julia
julia> using Genie
julia> Genie.loadapp()
```
The app's environment will now be loaded.
In order to start the web server execute
```julia
julia> up()
```
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 914 | # Managing external packages for your Genie app
Genie fully takes advantage of Julia's excellent package manager, `Pkg` -- while allowing Genie developers to use any
third party package available in Julia's ecosystem. This is achieved by taking a common sense approach: Genie apps are
just plain Julia projects.
In order to add extra packages to your Genie app, thus, we need to use Julia's `Pkg` features:
1. start a Genie REPL with your app: `$ bin/repl`. This will automatically load the package environment of the app.
2. switch to `Pkg` mode: `julia> ]`
3. add the package you want, for example `OhMyREPL`: `(MyGenieApp) pkg> add OhMyREPL`
That's all! Now you can use the packages at the Genie REPL or anywhere in your app via `using` or `import`.
Use the same approach to update the packages in your app, via: `pkg> up` and apply all available updates,
or `pkg> up OhMyREPL` to update a single package.
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 12013 | # Advanced routing techniques
Genie's router can be considered the brain of the app, matching web requests to handler functions, extracting and
setting up the request's variables and the execution environment, and invoking the response methods.
## Static routing
Starting with the simplest case, we can register "plain" routes by using the `route` method. The method takes as its
required arguments the URI string or pattern -- and the handler function that will be invoked in order to provide the
response. The router supports two ways of registering routes, either `route(pattern::String, f::Function)` or
`route(f::Function, pattern::String)`. The first syntax is for passing function references -- while the second is for
defining inline functions (lambdas).
### Example
The following snippet defines a function `greet` which returns the "Welcome to Genie!" string. We use the function as
our route handler, by passing a reference to it as the second argument to the `route` method.
```julia
using Genie
greet() = "Welcome to Genie!"
route("/greet", greet) # [GET] /greet => greet
up() # start the server
```
If you use your browser to navigate to <http://127.0.0.1:8000/greet> you'll see the code in action.
However, defining a dedicated handler function might be overkill for simple cases like this. As such, Genie allows
registering in-line handlers:
```julia
route("/bye") do
"Good bye!"
end # [GET] /bye => ()
```
You can just navigate to <http://127.0.0.1:8000/bye> -- the route is instantly available in the app.
---
**HEADS UP**
The routes are matched from newest to oldest. This means that you can define a new route to overwrite a previously defined one.
Genie's router won't match the most specific rule, but the first matching one. So if, for example, you register a route
to match `/*`, it will handle all the requests, even if you have previously defined more specific routes. As a side-note,
you can use this technique to temporarily divert all users to a maintenance page (which you can later remove by deleting
the route using `Router.delete!(:route_name)`).
---
## Dynamic routing (using route parameters)
Static routing works great for fixed URLs. But what if we have dynamic URLs, where the components map to information in
the backend (like database IDs) and vary with each request? For example, how would we handle a URL like
`"/customers/57943/orders/458230"`, where `57943` is the customer id and `458230` is the order id.
Such situations are handled through dynamic routing or route parameters. For the previous example,
`"/customers/57943/orders/458230"`, we can define a dynamic route as `"/customers/:customer_id/orders/:order_id"`.
Upon matching the request, the Router will unpack the values and expose them in the `params` collection.
### Example
```julia
using Genie, Genie.Requests
route("/customers/:customer_id/orders/:order_id") do
"You asked for the order $(payload(:order_id)) for customer $(payload(:customer_id))"
end
up()
```
## Routing methods (`GET`, `POST`, `PUT`, `PATCH`, `DELETE`, `OPTIONS`)
By default, routes handle `GET` requests, since these are the most common. In order to define routes for handling other
types of request methods, we need to pass the `method` keyword argument, indicating the HTTP method we want to respond to.
Genie's Router supports `GET`, `POST`, `PUT`, `PATCH`, `DELETE`, `OPTIONS` methods.
The router defines and exports constants for each of these as `Router.GET`, `Router.POST`, `Router.PUT`, `Router.PATCH`,
`Router.DELETE`, and `Router.OPTIONS`.
### Example
We can setup the following `PATCH` route:
```julia
using Genie, Genie.Requests
route("/patch_stuff", method = PATCH) do
"Stuff to patch"
end
up()
```
And we can test it using the `HTTP` package:
```julia
using HTTP
HTTP.request("PATCH", "http://127.0.0.1:8000/patch_stuff").body |> String
```
This will output the string "Stuff to patch", as the response from the `PATCH` request. By sending a request with the
`PATCH` method, our route is triggered. Consequently, we access the response body and convert it to a string, which is
"Stuff to patch".
## Named routes
Genie allows tagging routes with names. This is a very powerful feature, to be used in conjunction with the
`Router.tolink` method, for dynamically generating URLs to various the routes. The advantage of this technique is that
if we refer to the route by name and generate the links dynamically using `tolink`, as long as the name of the route
stays the same, if we change the route pattern, all the URLs will automatically match the new route definition.
In order to name a route we need to use the `named` keyword argument, which expects a `Symbol`.
### Example
```julia
using Genie, Genie.Requests
route("/customers/:customer_id/orders/:order_id", named = :get_customer_order) do
"Looking up order $(payload(:order_id)) for customer $(payload(:customer_id))"
end
# [GET] /customers/:customer_id/orders/:order_id => ()
```
We can check the status of our route with:
```julia
julia> routes()
:get_customer_order => [GET] /customers/:customer_id/orders/:order_id => ()
```
---
**HEADS UP**
For consistency, Genie names all the routes. However, the auto-generated name is state dependent. So if you change the
definition of the route, it's possible that the name will change as well. Thus, it's best to explicitly name the routes
if you plan on referencing them throughout the app.
---
We can confirm this by adding an anonymous route:
```julia
route("/foo") do
"foo"
end
# [GET] /foo => ()
julia> routes()
:get_customer_order => [GET] /customers/:customer_id/orders/:order_id => ()
:get_foo => [GET] /foo => ()
```
The new route has been automatically named `get_foo`, based on the method and URI pattern.
## Links to routes
We can use the name of the route to link back to it using the `linkto` method.
### Example
Let's start with the previously defined two routes:
```julia
julia> routes()
:get_customer_order => [GET] /customers/:customer_id/orders/:order_id => ()
:get_foo => [GET] /foo => ()
```
Static routes such as `:get_foo` are straightforward to target:
```julia
julia> linkto(:get_foo)
"/foo"
```
For dynamic routes, it's a bit more involved as we need to supply the values for each of the parameters, as keyword
arguments:
```julia
julia> linkto(:get_customer_order, customer_id = 1234, order_id = 5678)
```
This will generate the URL `"/customers/1234/orders/5678"`
The `linkto` function should be used in conjunction with the HTML code for generating links, ie:
```html
<a href="$(linkto(:get_foo))">Foo</a>
```
## Listing routes
At any time we can check which routes are registered with `Router.routes`:
```julia
julia> routes()
[GET] /foo => getfield(Main, Symbol("##7#8"))()
[GET] /customers/:customer_id/orders/:order_id => ()
```
### The `Route` type
The routes are represented internally by the `Route` type which has the following fields:
* `method::String` - for storing the method of the route (`GET`, `POST`, etc)
* `path::String` - represents the URI pattern to be matched against
* `action::Function` - the route handler to be executed when the route is matched
* `name::Union{Symbol,Nothing}` - the name of the route
* `context::Module` - an optional context to be used when executing the route handler
## Removing routes
We can delete routes from the stack by calling the `delete!` method and passing the collection of routes and the name
of the route to be removed. The method returns the collection of (remaining) routes
### Example
```julia
julia> routes()
:get_customer_order => [GET] /customers/:customer_id/orders/:order_id => ()
:get_foo => [GET] /foo => ()
julia> Router.delete!(:get_foo)
:get_customer_order => [GET] /customers/:customer_id/orders/:order_id => ()
julia> routes()
:get_customer_order => [GET] /customers/:customer_id/orders/:order_id => ()
```
## Matching routes by type of arguments
By default route parameters are parsed into the `payload` collection as `SubString{String}`:
```julia
using Genie, Genie.Requests
route("/customers/:customer_id/orders/:order_id") do
"Order ID has type $(payload(:order_id) |> typeof) // Customer ID has type $(payload(:customer_id) |> typeof)"
end
```
This will output `Order ID has type SubString{String} // Customer ID has type SubString{String}`
However, for such a case, we'd very much prefer to receive our data as `Int` to avoid an explicit conversion --
_and_ to match only numbers. Genie supports such a workflow by allowing type annotations to route parameters:
```julia
route("/customers/:customer_id::Int/orders/:order_id::Int", named = :get_customer_order) do
"Order ID has type $(payload(:order_id) |> typeof) // Customer ID has type $(payload(:customer_id) |> typeof)"
end
# [GET] /customers/:customer_id::Int/orders/:order_id::Int => ()
```
Notice how we've added type annotations to `:customer_id` and `:order_id` in the form `:customer_id::Int` and `:order_id::Int`.
However, attempting to access the URL `http://127.0.0.1:8000/customers/10/orders/20` will fail:
```julia
Failed to match URI params between Int64::DataType and 10::SubString{String}
MethodError(convert, (Int64, "10"), 0x00000000000063fe)
/customers/10/orders/20 404
```
As you can see, Genie attempts to convert the types from the default `SubString{String}` to `Int` -- but doesn't know how.
It fails, can't find other matching routes and returns a `404 Not Found` response.
### Type conversion in routes
The error is easy to address though: we need to provide a type converter from `SubString{String}` to `Int`.
```julia
Base.convert(::Type{Int}, v::SubString{String}) = parse(Int, v)
```
Once we register the converter our request will be correctly handled, resulting in `Order ID has type Int64 // Customer ID has type Int64`
## Matching individual URI segments
Besides matching the full route, Genie also allows matching individual URI segments. That is, enforcing that the various
route parameters obey a certain pattern. In order to introduce constraints for route parameters we append `#pattern` at
the end of the route parameter.
### Example
For instance, let's assume that we want to implement a localized website where we have a URL structure like: `mywebsite.com/en`,
`mywebsite.com/es` and `mywebsite.com/de`. We can define a dynamic route and extract the locale variable to serve localized content:
```julia
route(":locale", TranslationsController.index)
```
This will work very well, matching requests and passing the locale into our code within the `payload(:locale)` variable.
However, it will also be too greedy, virtually matching all the requests, including things like static files
(ie `mywebsite.com/favicon.ico`). We can constrain what the `:locale` variable can match, by appending the pattern
(a regex pattern):
```julia
route(":locale#(en|es|de)", TranslationsController.index)
```
The refactored route only allows `:locale` to match one of `en`, `es`, and `de` strings.
---
**HEADS UP**
Keep in mind not to duplicate application logic. For instance, if you have an array of supported locales, you can use that
to dynamically generate the pattern -- routes can be fully dynamically generated!
```julia
const LOCALE = ":locale#($(join(TranslationsController.AVAILABLE_LOCALES, '|')))"
route("/$LOCALE", TranslationsController.index, named = :get_index)
```
---
## The `params` collection
It's good to know that the router bundles all the parameters of the current request into the `params` collection
(a `Dict{Symbol,Any}`). This contains valuable information, such as route parameters, query params, POST payload, the
original `HTTP.Request` and `HTTP.Response` objects, etcetera. In general it's recommended not to access the `params`
collection directly but through the utility methods defined by `Genie.Requests` and `Genie.Responses` -- but knowing
about `params` might come in handy for advanced users.
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 2122 | # Customized application configuration with initializers
Initializers are plain Julia files which are loaded early in the application life-cycle (before routes, controller, or models).
They are designed to implement configuration code which is used by other parts of the application (like database connections,
logging settings, etc).
Initializers should be placed within the `config/initializers/` folder and they will be automatically loaded by Genie into the app.
**If your configuration is environment dependent (like a database connection which is different between dev and prod environments),
it should be added to the corresponding `config/env/*.jl` file.**
## Best practices
* You can name the initializers as you wish (ideally a descriptive name, like `redis.jl` for connecting to a Redis DB).
* Don't use uppercase names unless you define a module (in order to respect Julia's naming practices).
* Keep your initializer files small and focused, so they serve only one purpose.
* You can add as many initializers as you need.
* Do not abuse them, they are not meant to host complex code - app logic should be in models and controllers.
## Load order
The initializers are loaded in the order they are read from the file system. If you have initializers which depend on
other initializers, this is most likely a sign that you need to refactor using a model or a library file.
---
**HEADS UP**
Library files are Julia files which provide distinct functionality and can be placed in the `lib/` folder where they are
also automatically loaded by Genie. If the `lib/` folder does not exist, you can create it yourself.
---
## Scope
All the definitions (variables, constants, functions, modules, etc) added to initializer files are loaded into your
app's module. So if your app is called `MyGenieApp`, the definitions will be available under the `MyGenieApp` module.
---
**HEADS UP**
Given that your app's name is variable, you can also access your app's module through the `Main.UserApp` constant.
So all the definitions added to initializers can also be accessed through the `Main.UserApp` module.
---
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 836 | # The secrets (`config/secrets.jl`) file
Confidential configuration data (like API keys, usernames, passwords, etc) should be added to the `config/secrets.jl` file.
This file is by default added to `.gitignore` when creating a Genie app, so it won't be added to source control --
to avoid that it is accidentally exposed.
## Scope
All the definitions (variables, constants, functions, modules, etc) added to the `secrets.jl` file are loaded into your
app's module. So if your app (and its main module) is called `MyGenieApp`, the definitions will be available under the `MyGenieApp` namespace.
---
**HEADS UP**
Given the your app's name is variable, you can also access your app's module through the `Main.UserApp` constant. So all
the definitions added to `secrets.jl` can also be accessed through the `Mani.UserApp` module.
---
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 517 | # The `lib/` folder
Genie makes it easy to automatically load Julia code (modules, files, etc) into an app, outside of the standard Genie
MVC app structure. You simply need to add your files and folders into the `lib/` folder.
---
**HEADS UP**
* If the `lib/` folder does not exist, just create it yourself: `julia> mkdir("lib")`
* Genie includes the files placed within the `lib/` folder and subfolders _recursively_
* Files within `lib/` are loaded using `Revise` and are automatically reloaded if changed.
---
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 7087 | # Using Genie with Docker
Genie comes with extended support for containerizing apps using Docker. The functionality is provided by the official
`GenieDeployDocker` plugin.
## Setting up `GenieDeployDocker`
In order to use the Docker integration features, first we need to add the `GenieDeployDocker` plugin for `Genie`.
```julia
pkg> add GenieDeployDocker
```
## Generating the Genie-optimised `Dockerfile`
You can bootstrap the Docker setup by invoking the `GenieDeployDocker.dockerfile()` function. This will generate a
custom `Dockerfile` optimized for Genie web apps containerization. The file will be generated in the current work dir
(or where instructed by the optional argument `path` -- see the help for the `dockerfile()` function).
Once generated, you can edit it and customize it as needed - Genie will not overwrite the file, thus preserving any changes
(unless you call the `dockerfile` function again, passing the `force=true` argument).
The behavior of `dockerfile()` can be controlled by passing any of the multiple optional arguments supported.
## Building the Docker container
Once we have our `Dockerfile` ready, we can invoke `GenieDeployDocker.build()` to set up the Docker container. You can
pass any of the supported optional arguments to configure settings such as the container's name (by default `"genie"`),
the path (defaults to current work dir), and others (see the output of `help?> GenieDeployDocker.dockerfile` for all the
available options).
## Running the Genie app within the Docker container
When the image is ready, we can run it with `GenieDeployDocker.run()`. We can configure any of the optional arguments in
order to control how the app is run. Check the inline help for the function for more details.
## Examples
First let's create a Genie app:
```julia
julia> using Genie
julia> Genie.Generator.newapp("DockerTest")
[ Info: Done! New app created at /your/app/path/DockerTest
# output truncated
```
When it's ready, let's add the `Dockerfile`:
```julia
julia> using GenieDeployDocker
julia> GenieDeployDocker.dockerfile()
Docker file successfully written at /your/app/path/DockerTest/Dockerfile
```
Now, to build our container:
```julia
julia> GenieDeployDocker.build()
# output truncated
Successfully tagged genie:latest
Docker container successfully built
```
And finally, we can now run our app within the Docker container:
```julia
julia> GenieDeployDocker.run()
Starting docker container with `docker run -it --rm -p 80:8000 --name genieapp genie bin/server`
# output truncated
```
We should then see the familiar Genie loading screen, indicating the app's loading progress and notifying us once the app is running.
Our application starts inside the Docker container, binding port 8000 within the container (where the Genie app is running)
to the port 80 of the host. So we are now able to access our app at `http://localhost`. If you navigate to `http://localhost` w
ith your favorite browser you'll see Genie's welcome page. Notice that we don't access on port 8000 - this page is served
from the Docker container on the default port 80.
## Inspecting the containers
We can get a list of available container by using `GenieDeployDocker.list()`. This will show only the currently running containers
by default, but we can pass the `all=true` argument to also include containers that are offline.
```julia
julia> GenieDeployDocker.list()
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
c87bfd8322cc genie "bin/server" 6 minutes ago Up 6 minutes 80/tcp, 0.0.0.0:80->8000/tcp genieapp
Process(`docker ps`, ProcessExited(0))
```
## Stopping running containers
The running containers can be stopped by using the `GenieDeployDocker.stop()` function, passing the name of the container.
```julia
julia> GenieDeployDocker.stop("genieapp")
```
## Using Docker during development
If we want to use Docker to serve the app during development, we need to _mount_ our app from host (your computer) into
the container -- so that we can keep editing our files locally, but see the changes reflected in the Docker container.
In order to do this we need to pass the `mountapp = true` argument to `GenieDeployDocker.run()`, like this:
```julia
julia> GenieDeployDocker.run(mountapp = true)
Starting docker container with `docker run -it --rm -p 80:8000 --name genieapp -v /Users/adrian/DockerTest:/home/genie/app genie bin/server`
```
When the app finishes starting, we can edit the files on the host using our favorite IDE, and see the changes reflected
in the Docker container.
## Creating an optimized Genie sysimage with `PackageCompiler.jl`
If we are using Docker containers to deploy Genie apps in production, you can greatly improve the performance of the app
by preparing a precompiled sysimage for Julia. We can include this workflow as part of the Docker `build` step as follows.
### Edit the `Dockerfile`
We'll start by making a few changes to our `Dockerfile`, as follows:
1/ Under the line `WORKDIR /home/genie/app` add
```dockerfile
# C compiler for PackageCompiler
RUN apt-get update && apt-get install -y g++
```
2/ Under the line starting with `RUN julia -e` add
```dockerfile
# Compile app
RUN julia --project compiled/make.jl
```
You may also want to replace the line saying
```dockerfile
ENV GENIE_ENV "dev"
```
with
```dockerfile
ENV GENIE_ENV "prod"
```
to configure the application to run in production (first test locally to make sure that everything is properly configured
to run the app in production environment).
### Add `PackageCompiler.jl`
We also need to add `PackageCompiler` as a dependency of our app:
```julia
pkg> add PackageCompiler
```
### Add the needed files
Create a new folder to host our files:
```julia
julia> mkdir("compiled")
```
Now create the following files:
```julia
julia> touch("compiled/make.jl")
julia> touch("compiled/packages.jl")
```
### Edit the files
Now to put the content into each of the files.
#### Preparing the `packages.jl` file
Here we simply put an array of package that our app uses and that we want to precompile, ex:
```julia
# packages.jl
const PACKAGES = [
"Dates",
"Genie",
"Inflector",
"Logging"
]
```
#### Preparing the `make.jl` file
Now edit the `make.jl` file as follows:
```julia
# make.jl
using PackageCompiler
include("packages.jl")
PackageCompiler.create_sysimage(
PACKAGES,
sysimage_path = "compiled/sysimg.so",
cpu_target = PackageCompiler.default_app_cpu_target()
)
```
#### Using the precompiled image
The result of these changes is that `PackageCompiler` will create a new Julia sysimage that will be stored inside the
`compiled/sysimg.so` file. The last step is to instruct our `bin/server` script to use the image.
Edit the `bin/server` file and make it look like this:
```bash
julia --color=yes --depwarn=no --project=@. --sysimage=compiled/sysimg.so -q -i -- $(dirname $0)/../bootstrap.jl -s=true "$@"
```
With this change we're passing the additional `--sysimage` flag, indicating our new Julia sys image. | Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 5494 | # Working with Web Sockets
Genie provides a powerful workflow for client-server communication over websockets. The system hides away the complexity
of the network level communication, exposing powerful abstractions which resemble Genie's familiar MVC workflow: the
clients and the server exchange messages over `channels` (which are the equivalent of `routes`).
## Registering `channels`
The messages are mapped to a matching channel, where are processed by Genie's `Router` which extracts the payload and
invokes the designated handler (controller method or function). For most purposes, the `channels` are the functional
equivalents of `routes` and are defined in a similar way:
```julia
using Genie.Router
channel("/foo/bar") do
# process request
end
channel("/baz/bax", YourController.your_handler)
```
The above `channel` definitions will handle websocket messages sent to `/foo/bar` and `/baz/bax`.
## Setting up the client
In order to enable WebSockets communication in the browser we need to load a JavaScript file. This is provided by Genie,
through the `Assets` module. Genie makes it extremely easy to setup the WebSockets infrastructure on the client side,
by providing the `Assets.channels_support()` method. For instance, if we want to add support for WebSockets to the root
page of a web app, all we need is this:
```julia
using Genie.Router, Genie.Assets
route("/") do
Assets.channels_support()
end
```
That is all we need in order to be able to push and receive messages between client and server.
---
## Try it!
You can follow through by running the following Julia code in a Julia REPL:
```julia
using Genie, Genie.Router, Genie.Assets
Genie.config.websockets_server = true # enable the websockets server
route("/") do
Assets.channels_support()
end
up() # start the servers
```
Now if you visit <http://localhost:8000> you'll get a blank page -- which, however, includes all the necessary
functionality for WebSockets communication! If you use the browser's developer tools, the Network pane will indicate
that a `channels.js` file was loaded and that a WebSockets request was made (Status 101 over GET). Additionally, if you
peek at the Console, you will see a `Subscription ready` message.
**What happened?**
At this point, by invoking `Assets.channels_support()`, Genie has done the following:
* loaded the bundled `channels.js` file which provides a JS API for communicating over WebSockets
* has created two default channels, for subscribing and unsubscribing: `/____/subscribe` and `/____/unsubscribe`
* has invoked `/____/subscribe` and created a WebSockets connection between client and server
### Pushing messages from the server
We are ready to interact with the client. Go to the Julia REPL running the web app and run:
```julia
julia> Genie.WebChannels.connected_clients()
1-element Array{Genie.WebChannels.ChannelClient,1}:
Genie.WebChannels.ChannelClient(HTTP.WebSockets.WebSocket{HTTP.ConnectionPool.Transaction{Sockets.TCPSocket}}(T0 🔁 0↑🔒 0↓🔒 100s 127.0.0.1:8001:8001 ≣16, 0x01, true, UInt8[0x7b, 0x22, 0x63, 0x68, 0x61, 0x6e, 0x6e, 0x65, 0x6c, 0x22 … 0x79, 0x6c, 0x6f, 0x61, 0x64, 0x22, 0x3a, 0x7b, 0x7d, 0x7d], UInt8[], false, false), ["____"])
```
We have one connected client to the `____` channel! We can send it a message:
```julia
julia> Genie.WebChannels.broadcast("____", "Hey!")
true
```
If you look in the browser's console you will see the "Hey!" message! By default, the client side handler simply outputs
the message. We're also informed that we can "Overwrite window.parse_payload to handle messages from the server".
Let's do it. Run this in the current REPL (it will overwrite our root route handler):
```julia
route("/") do
Assets.channels_support() *
"""
<script>
window.parse_payload = function(payload) {
console.log('Got this payload: ' + payload);
}
</script>
"""
end
```
Now if you reload the page and broadcast the message, it will be picked up by our custom payload handler.
We can remove clients that are no longer reachable (for instance, if the browser tab is closed) with:
```julia
julia> Genie.WebChannels.unsubscribe_disconnected_clients()
```
The output of `unsubscribe_disconnected_clients()` is the collection of remaining (connected) clients.
---
**Heads up!**
You should routinely `unsubscribe_disconnected_clients()` to free memory.
---
At any time, we can check the connected clients with `Genie.WebChannels.connected_clients()` and the disconnected ones
with `Genie.WebChannels.disconnected_clients()`.
### Pushing messages from the client
We can also push messages from client to server. As we don't have a UI, we'll use the browser's console and Genie's JavaScript
API to send the messages. But first, we need to set up the `channel` which will receive our message. Run this in the active Julia REPL:
```julia
channel("/____/echo") do
@info "Received: $(params(:payload))"
end
```
Now that our endpoint is up, go to the browser's console and run:
```javascript
Genie.WebChannels.sendMessageTo('____', 'echo', 'Hello!')
```
The julia terminal and console will both immediately display the response from the server:
```text
Received: Hello!
Got this payload: Received: Hello!
```
## Summary
This concludes our intro to working with WebSockets in Genie. You now have the knowledge to set up the communication between
client and server, send messages from both server and clients, and perform various tasks using the `WebChannels` API.
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 412 | # How to Install Genie
Install Genie from Julia's registry -- for example the latest version (currently version 5):
```julia
pkg> add Genie
```
Genie, just like Julia, uses semantic versioning in the form vX.Y.Z to designate:
- X : major version, introducing breaking changes
- Y : minor version, brings new features, no breaking changes
- Z : patch version, fixes bugs, no new features or breaking changes
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 3780 | # Hello world with Genie
Here are a few examples to quickly get you started with building Genie web apps.
## Running Genie interactively at the REPL or in notebooks
The simplest use case is to configure a routing function at the REPL and start the web server. That's all that's
needed to run your code on the web page:
### Example
```julia
julia> using Genie
julia> route("/hello") do
"Hello World"
end
julia> up()
```
The `route` function defines a mapping between a URL (`"/hello"`) and a Julia function (a _handler_) which will be
automatically invoked to send the response back to the client. In this case we're sending back the string "Hello World".
That's all! We have set up an app, a route, and started the web server. Open your favorite web browser and go to
<http://127.0.0.1:8000/hello> to see the result.
---
**HEADS UP**
Keep in mind that Julia JIT-compiles. A function is automatically compiled the first time it is invoked. The function,
in this case, is our route handler that is responding to the request. This will make the first response slower as it
also includes compilation time. But once the function is compiled, for all the subsequent requests, it will be super fast!
---
## Developing a simple Genie script
Genie can also be used in custom scripts, for example when building micro-services with Julia.
Let's create a simple "Hello World" micro-service.
Start by creating a new file to host our code -- let's call it `geniews.jl`
```julia
julia> touch("geniews.jl")
```
Now, open it in the editor:
```julia
julia> edit("geniews.jl")
```
Add the following code:
```julia
using Genie, Genie.Renderer, Genie.Renderer.Html, Genie.Renderer.Json
route("/hello.html") do
html("Hello World")
end
route("/hello.json") do
json("Hello World")
end
route("/hello.txt") do
respond("Hello World", :text)
end
up(8001, async = false)
```
We begun by defining 2 routes and we used the `html` and `json` rendering functions (available in the `Renderer.Html`
and the `Renderer.Json` modules). These functions are responsible for outputting the data using the correct format and
document type (with the correct MIME), in our case HTML data for `hello.html`, and JSON data for `hello.json`.
The third `route` serves text responses. As Genie does not provide a specialized `text()` method for sending `text/plain`
responses, we use the generic `respond` function, indicating the desired MIME type. In our case `:text`, corresponding
to `text/plain`. Other available MIME types shortcuts are `:xml`, `:markdown`, `:javascript` and a few others others --
and users can register their own mime types and response types as needed or can pass the full mime type as a string,
ie `"text/csv"`.
The `up` function will launch the web server on port `8001`. This time, very important, we instructed it to start the
server synchronously (that is, _blocking_ the execution of the script), by passing the `async = false` argument.
This way we make sure that our script stays running. Otherwise, at the end of the script, the Julia process would normally exit,
killing our server.
In order to launch the script, run `$ julia geniews.jl`.
## Batteries included
Genie readily makes available a rich set of features - you have already seen the rendering and the routing modules in
action. But for instance, logging (to file and console) can also be easily triggered with one line of code, powerful
caching can be enabled with a couple more lines, and so on.
The app already handles "404 Page Not Found" and "500 Internal Error" responses. If you try to access a URL which is not
handled by the app, like say <http://127.0.0.1:8001/not_here>, you'll see Genie's default 404 page. The default error
pages can be overwritten with custom ones.
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 4586 | # Developing Genie Web Services
Starting up ad-hoc web servers at the REPL and writing small scripts to wrap micro-services works great, but production
apps tend to become complex quickly. They also have more stringent requirements, like managing dependencies, compressing
assets, reloading code, logging, environments, or structuring the codebase in a way which promotes efficient workflows
when working in teams.
Genie enables a modular approach towards app building, allowing to add more components as the need arises. You can start
with the web service template (which includes dependencies management, logging, environments, and routing), and grow it
by sequentially adding DB persistence (through the SearchLight ORM), high performance HTML view templates with embedded
Julia (via `Renderer.Html`), caching, authentication, and more.
## Setting up a Genie Web Service project
Genie packs handy generator features and templates which help bootstrapping and setting up various parts of an application.
These are available in the `Genie.Generator` module.
For bootstrapping a new app we need to invoke one of the functions in the `newapp` family:
```julia
julia> using Genie
julia> Genie.Generator.newapp_webservice("MyGenieApp")
```
If you follow the log messages in the REPL you will see that the command will trigger a flurry of actions in order to set up the new project:
- it creates a new folder, `MyGenieApp/`, which will hosts the files of the app and whose name corresponds to the name of the app,
- within the `MyGenieApp/` folder, it creates the files and folders needed by the app,
- changes the active directory to `MyGenieApp/` and creates a new Julia project within it (adding the `Project.toml` file),
- installs all the required dependencies for the new Genie app (using `Pkg` and the standard `Manifest.toml` file), and finally,
- starts the web server
---
**TIP**
Check out the `?help` documentation for `Genie.Generator.newapp`, `Genie.Generator.newapp_webservice`,
`Genie.Generator.newapp_mvc`, and `Genie.Generator.newapp_fullstack` too see what options are available for bootstrapping
applications. We'll go over the different configurations in upcoming sections.
---
## The file structure
Our newly created web service has this file structure:
```julia
├── .gitattributes
├── .gitignore
├── Manifest.toml
├── Project.toml
├── bin
├── bootstrap.jl
├── config
├── public
├── routes.jl
├── src
└── test
```
These are the roles of each of the files and folders:
- `Manifest.toml` and `Project.toml` are used by Julia and `Pkg` to manage the app's dependencies.
- `bin/` includes scripts for starting up a Genie REPL or a Genie server.
- `bootstrap.jl` and the files within `src/` are used by Genie to load the application and _should not be modified_ unless you know what you're doing.
- `config/` includes the per-environment configuration files.
- `public/` is the document root, which includes static files exposed by the app on the network/internet.
- `routes.jl` is the dedicated file for registering Genie routes.
- the `test/` folder is set up to store the unit and integration tests for the app.
- `.gitattributes` and `.gitignore` are used by Git to manage the project's files.
---
**HEADS UP**
After creating a new app you might need to change the file permissions to allow editing/saving the files such as `routes.jl`.
---
## Adding logic
You can now edit the `routes.jl` file to add some logic, at the bottom of the file:
```julia
route("/hello") do
"Welcome to Genie!"
end
```
If you now visit <http://127.0.0.1:8000/hello> you'll see a warm greeting.
## Extending the app
Genie apps are just plain Julia projects. This means that `routes.jl` will behave like any other Julia script - you can
reference extra packages, you can switch into `pkg>` mode to manage per project dependencies, include other files, etcetera.
If you have existing Julia code that you want to quickly load into a Genie app, you can add a `lib/` folder in the root
of the app and place your Julia files there. If the folder exists, `lib/` and all its subfolders are automatically loaded
by Genie, recursively.
---
**WARNING**
If you add the `lib/` folder while the Genie app is running, you will need to restart the app to load the files.
---
If you need to add database support, you can always add the SearchLight ORM by running `julia> Genie.Generator.db_support()`
in the app's REPL.
However, if your app grows in complexity and you develop it from scratch, it is more efficient to take advantage of
Genie's resource-oriented MVC structure.
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 14941 | we'# Developing Genie MVC Apps
Here is a complete walk-through of developing a feature rich MVC app with Genie, including both user facing web pages,
a REST API endpoint, and user authentication.
---
## Getting started - creating the app
First, let's create a new Genie MVC app. We'll use Genie's app generator, so first let's make sure we have Genie installed.
Let's start a Julia REPL and add Genie:
```julia
pkg> add Genie # press ] from julia> prompt to enter Pkg mode
```
Now, to create the app:
```julia
julia> using Genie
julia> Genie.Generator.newapp_mvc("Watch Tonight")
```
---
**HEADS UP**
The `newapp_mvc` function creates a new app with the given name, although spaces are not allowed in the name and Genie
will automatically correct it as `WatchTonight`. `WatchTonight` is the name of the app and the name of the main module of
the project, in the `src/` folder. You will see it used when we will reference the various files in the project, in the
`using` statements.
---
Genie will bootstrap a new application for us, creating the necessary files and installing dependencies. As we're
creating a MVC app, Genie will offer to install support for SearchLight, Genie's ORM, and will ask what database backend
we'll want to use:
```shell
Please choose the DB backend you want to use:
1. SQLite
2. MySQL
3. PostgreSQL
Input 1, 2 or 3 and press ENTER to confirm
```
Note that if you select an option other than SQLite, you will need to manually create the database outside of Genie.
Currently, Genie only automatically creates SQLite databases.
We'll use SQLite in this demo, so let's press "1". Once the process is completed, Genie will start the new application
at <http://127.0.0.1:8000>. We can open it in the browser to see the default Genie home page.
### How does this work?
Genie uses the concept of routes and routing in order to map a URL to a request handler (a Julia function) within the app.
If we edit the `routes.jl` file we will see that is has defined a `route` that states that for any requests to the `/` URL,
the app will display a static file called `welcome.html` (which can be found in the `public/` folder):
```julia
route("/") do
serve_static_file("welcome.html")
end
```
## Connecting to the database
In order to configure the database connection we need to edit the `db/connection.yml` file, to make it look like this:
```yaml
env: ENV["GENIE_ENV"]
dev:
adapter: SQLite
database: db/netflix_catalog.sqlite
```
Now let's manually load the database configuration:
```julia
julia> include(joinpath("config", "initializers", "searchlight.jl"))
```
## Creating a Movie resource
A resource is an entity exposed by the application at a URL. In a Genie MVC app it represents a bundle of Model, views,
and Controller files - as well as possible additional files such as migration files for creating a database table, tests,
a model data validator, etc.
In the REPL run:
```julia
julia> Genie.Generator.newresource("movie")
julia> using SearchLight
julia> SearchLight.Generator.newresource("movie")
```
This should create a series of files to represent the Movie resource - just take a look at the output to see what and
where was created.
### Creating the DB table using the database migration
We need to edit the migrations file that was just created in `db/migrations/`. Look for a file that ends in
`_create_table_movies.jl` and make it look like this:
```julia
module CreateTableMovies
import SearchLight.Migrations: create_table, column, primary_key, add_index, drop_table
function up()
create_table(:movies) do
[
primary_key()
column(:type, :string, limit = 10)
column(:title, :string, limit = 100)
column(:directors, :string, limit = 100)
column(:actors, :string, limit = 250)
column(:country, :string, limit = 100)
column(:year, :integer)
column(:rating, :string, limit = 10)
column(:categories, :string, limit = 100)
column(:description, :string, limit = 1_000)
]
end
add_index(:movies, :title)
add_index(:movies, :actors)
add_index(:movies, :categories)
add_index(:movies, :description)
end
function down()
drop_table(:movies)
end
end
```
#### Creating the migrations table
In order to be able to manage the app's migrations, we need to create the DB table used by SearchLight's migration system.
This is easily done using SearchLight's generators:
```julia
julia> SearchLight.Migration.init()
```
### Running the migration
We can now check the status of the migrations:
```julia
julia> SearchLight.Migration.status()
```
We should see that we have one migration that is `DOWN` (meaning that we need to run the migration because it has not been
executed yet).
We execute the migration by running the last migration UP:
```julia
julia> SearchLight.Migration.lastup()
```
If you now recheck the status of the migrations, you should see that the migration is now `UP`.
## Creating the Movie model
Now that we have the database table, we need to create the model file which allows us manage the data. The file has
already been created for us in `app/resources/movies/Movies.jl`. Edit it and make it look like this:
```julia
module Movies
import SearchLight: AbstractModel, DbId
import Base: @kwdef
export Movie
@kwdef mutable struct Movie <: AbstractModel
id::DbId = DbId()
type::String = "Movie"
title::String = ""
directors::String = ""
actors::String = ""
country::String = ""
year::Int = 0
rating::String = ""
categories::String = ""
description::String = ""
end
end
```
### Interacting with the movies data
Once our model is created, we can interact with the database:
```julia
julia> using Movies
julia> m = Movie(title = "Test movie", actors = "John Doe, Jane Doe")
```
We can check if our movie object is persisted (saved to the db):
```julia
julia> ispersisted(m)
false
```
And we can save it:
```julia
julia> save(m)
true
```
Now we can run various methods against our data:
```julia
julia> count(Movie)
julia> all(Movie)
```
### Seeding the data
We're now ready to load the movie data into our database - we'll use a short seeding script. First make sure to place
the CVS file into the `/db/seeds/` folder. Create the seeds file:
```julia
julia> touch(joinpath("db", "seeds", "seed_movies.jl"))
```
And edit it to look like this:
```julia
using SearchLight, WatchTonight.Movies
using CSV
Base.convert(::Type{String}, _::Missing) = ""
Base.convert(::Type{Int}, _::Missing) = 0
Base.convert(::Type{Int}, s::String) = parse(Int, s)
function seed()
for row in CSV.Rows(joinpath(@__DIR__, "netflix_titles.csv"), limit = 1_000)
m = Movie()
m.type = row.type
m.title = row.title
m.directors = row.director
m.actors = row.cast
m.country = row.country
m.year = parse(Int, row.release_year)
m.rating = row.rating
m.categories = row.listed_in
m.description = row.description
save(m)
end
end
```
Add CSV.jl as a dependency of the project:
```julia
pkg> add CSV
```
And download the dataset:
```julia
julia> download("https://raw.githubusercontent.com/essenciary/genie-watch-tonight/main/db/seeds/netflix_titles.csv", joinpath("db", "seeds", "netflix_titles.csv"))
```
Now, to seed the db:
```julia
julia> include(joinpath("db", "seeds", "seed_movies.jl"))
julia> seed()
```
## Setting up the web page
We'll start by adding the route to our handler function. Let's open the `routes.jl` file and add:
```julia
# routes.jl
using WatchTonight.MoviesController
route("/movies", MoviesController.index)
```
This route declares that the `/movies` URL will be handled by the `MoviesController.index` index function. Let's put it
in by editing `/app/resources/movies/MoviesController.jl`:
```julia
module MoviesController
function index()
"Welcome to movies list!"
end
end
```
If we navigate to <http://127.0.0.1:8000/movies> we should see the welcome.
Let's make this more useful though and display a random movie upon landing here:
```julia
module MoviesController
using Genie.Renderer.Html, SearchLight, WatchTonight.Movies
function index()
html(:movies, :index, movies = rand(Movie))
end
end
```
The index function renders the `/app/resources/movies/views/index.jl.html` view file as HTML, passing it a random movie
into the `movies` instance. Since we don't have the view file yet, let's add it:
```julia
julia> touch(joinpath("app", "resources", "movies", "views", "index.jl.html"))
```
Make it look like this:
```html
<h1 class="display-1 text-center">Watch tonight</h1>
<%
if ! isempty(movies)
for_each(movies) do movie
partial(joinpath(Genie.config.path_resources, "movies", "views", "_movie.jl.html"), movie = movie)
end
else
partial(joinpath(Genie.config.path_resources, "movies", "views", "_no_results.jl.html"))
end
%>
```
Now to create the `_movie.jl.html` partial file to render a movie object:
```julia
julia> touch(joinpath("app", "resources", "movies", "views", "_movie.jl.html"))
```
Edit it like this:
```html
<div class="container" style="margin-top: 40px;">
<h3><% movie.title %></h3>
<div>
<small class="badge bg-primary"><% movie.year %></small> |
<small class="badge bg-light text-dark"><% movie.type %></small> |
<small class="badge bg-dark"><% movie.rating %></small>
</div>
<h4><% movie.description %></h4>
<div><strong>Directed by: </strong><% movie.directors %></div>
<div><strong>Cast: </strong><% movie.actors %></div>
<div><strong>Country: </strong><% movie.country %></div>
<div><strong>Categories: </strong><% movie.categories %></div>
</div>
```
And finally, the `_no_results.jl.html` partial:
```julia
julia> touch(joinpath("app", "resources", "movies", "views", "_no_results.jl.html"))
```
Which must look like this:
```html
<h4 class="container">
Sorry, no results were found for "$(params(:search_movies))"
</h4>
```
### Using the layout file
Let's make the web page nicer by loading the Twitter Bootstrap CSS library. As it will be used across all the pages of
the website, we'll load it in the main layout file. Edit `/app/layouts/app.jl.html` to look like this:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<title>Genie :: The Highly Productive Julia Web Framework</title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5/dist/css/bootstrap.min.css" rel="stylesheet">
</head>
<body>
<div class="container">
<%
@yield
%>
</div>
</body>
</html>
```
## Adding the search feature
Now that we can display titles, it's time to implement the search feature. We'll add a search form onto our page.
Edit `/app/resources/movies/views/index.jl.html` to look like this:
```html
<h1 class="display-1 text-center">Watch tonight</h1>
<div class="container" style="margin-top: 40px;">
<form action="$( Genie.Router.linkto(:search_movies) )">
<input class="form-control form-control-lg" type="search" name="search_movies" placeholder="Search for movies and TV shows" />
</form>
</div>
<%
if ! isempty(movies)
for_each(movies) do movie
partial(joinpath(Genie.config.path_resources, "movies", "views", "_movie.jl.html"), movie = movie)
end
else
partial(joinpath(Genie.config.path_resources, "movies", "views", "_no_results.jl.html"))
end
%>
```
We have added a HTML `<form>` which submits a query term over GET.
Next, add the route:
```julia
route("/movies/search", MoviesController.search, named = :search_movies)
```
And the `MoviesController.search` function after updating the `using` section:
```julia
using Genie, Genie.Renderer, Genie.Renderer.Html, SearchLight, WatchTonight.Movies
function search()
isempty(strip(params(:search_movies))) && redirect(:get_movies)
movies = find(Movie,
SQLWhereExpression("title LIKE ? OR categories LIKE ? OR description LIKE ? OR actors LIKE ?",
repeat(['%' * params(:search_movies) * '%'], 4)))
html(:movies, :index, movies = movies)
end
```
Time to check our progress: <http://127.0.0.1:8000/movies>
## Building the REST API
Let's start by adding a new route for the API search:
```julia
route("/movies/search_api", MoviesController.search_api)
```
With the corresponding `search_api` method in the `MoviesController` model:
```julia
using Genie, Genie.Renderer, Genie.Renderer.Html, SearchLight, WatchTonight.Movies, Genie.Renderer.Json
function search_api()
movies = find(Movie,
SQLWhereExpression("title LIKE ? OR categories LIKE ? OR description LIKE ? OR actors LIKE ?",
repeat(['%' * params(:search_movies) * '%'], 4)))
json(Dict("movies" => movies))
end
```
## Bonus
Genie makes it easy to add database backed authentication for restricted area of a website, by using the
`GenieAuthentication` plugin. Start by adding package:
```julia
pkg> add GenieAuthentication
julia> using GenieAuthentication
```
Now, to install the plugin files:
```julia
julia> GenieAuthentication.install(@__DIR__)
```
The plugin has created a create table migration that we need to run `UP`:
```julia
julia> SearchLight.Migration.up("CreateTableUsers")
```
Let's generate an Admin controller that we'll want to protect by login:
```julia
julia> Genie.Generator.newcontroller("Admin", pluralize = false)
```
Let's load the plugin into the app manually to avoid restarting the app. Upon restarting the application next time,
the plugin will be automatically loaded by `Genie`:
```julia
julia> include(joinpath("plugins", "genie_authentication.jl"))
```
Time to create an admin user for logging in:
```julia
julia> using WatchTonight.Users
julia> u = User(email = "admin@admin", name = "Admin", password = Users.hash_password("admin"), username = "admin")
julia> save!(u)
```
We'll also need a route for the admin area:
```julia
using WatchTonight.AdminController
route("/admin/movies", AdminController.index, named = :get_home)
```
And finally, the controller code:
```julia
module AdminController
using GenieAuthentication, Genie.Renderer, Genie.Exceptions, Genie.Renderer.Html
function index()
authenticated!()
h1("Welcome Admin") |> html
end
end
```
If we navigate to `http://127.0.0.1:8000/admin/movies` we'll be asked to logged in. Using `admin` for the user and
`admin` for the password will allow us to access the password protected section.
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 1632 | # Handling query params (GET variables)
Genie makes it easy to access query params, which are values sent as part of the URL over GET requests
(ex: mywebsite.com/index?foo=1&bar=2 `foo` and `bar` are query params corresponding to the variables `foo = 1` and `bar = 2`).
All these values are automatically collected by Genie and exposed in the `params()` collection (which is part of the `Router` module).
### Example
```julia
using Genie
route("/hi") do
name = params(:name, "Anon")
"Hello $name"
end
```
If you access <http://127.0.0.1:8000/hi> the app will respond with "Hello Anon" since we're not passing any query params.
However, requesting <http://127.0.0.1:8000/hi?name=Adrian> will in turn display "Hello Adrian" as we're passing the
`name` query variable with the value `Adrian`. This variable is exposed by Genie as `params(:name)`.
Genie however provides utility methods for accessing these values in the `Requests` module.
## The `Requests` module
Genie provides a set of utilities for working with requests data within the `Requests` module. You can use the
`getpayload` method to retrieve the query params as a `Dict{Symbol,Any}`. We can rewrite the previous route to take
advantage of the `Requests` utilities.
### Example
```julia
using Genie, Genie.Requests
route("/hi") do
"Hello $(getpayload(:name, "Anon"))"
end
```
The `getpayload` function has a few specializations, and one of them accepts the key and a default value. The default
value is returned if the `key` variable is not defined. You can see the various implementations for `getpayload` using
the API docs or Julia's `help>` mode.
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 1314 | # Reading POST payloads
Genie makes it easy to work with `POST` data. First, we need to register a dedicated route to handle `POST` requests.
Then, when a `POST` request is received, Genie will automatically extract the payload, making it accessible throughout
the `Requests.postpayload` method -- and appending it to the `Router.params(:POST)` collection.
## Handling `form-data` payloads
The following snippet registers two routes in the root of the app, one for `GET` and the other for `POST` requests.
The `GET` route displays a form which submits over `POST` to the other route. Finally, upon receiving the data,
we display a message.
### Example
```julia
using Genie, Genie.Renderer.Html, Genie.Requests
form = """
<form action="/" method="POST" enctype="multipart/form-data">
<input type="text" name="name" value="" placeholder="What's your name?" />
<input type="submit" value="Greet" />
</form>
"""
route("/") do
html(form)
end
route("/", method = POST) do
"Hello $(postpayload(:name, "Anon"))"
end
up()
```
The `postpayload` function has a few specializations, and one of them accepts the key and the default value. The default
value is returned if the `key` variable is not defined. You can see the various implementations for `postpayload` using
the API docs or Julia's `help>` mode.
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 1539 | # Using JSON payloads
A very common design pattern, especially when developing REST APIs, is to accept JSON payloads sent as `application/json`
data over `POST` requests. Genie efficiently handles this use case through the utility function `Requests.jsonpayload`.
Under the cover, Genie will process the `POST` request and will attempt to parse the JSON text payload. If this fails,
we can still access the raw data (the text payload not converted to JSON) by using the `Requests.rawpayload` method.
### Example
```julia
using Genie, Genie.Requests, Genie.Renderer.Json
route("/jsonpayload", method = POST) do
@show jsonpayload()
@show rawpayload()
json("Hello $(jsonpayload()["name"])")
end
up()
```
Next we make a `POST` request using the `HTTP` package:
```julia
using HTTP
HTTP.request("POST", "http://localhost:8000/jsonpayload", [("Content-Type", "application/json")], """{"name":"Adrian"}""")
```
We will get the following output:
```julia
jsonpayload() = Dict{String,Any}("name"=>"Adrian")
rawpayload() = "{\"name\":\"Adrian\"}"
INFO:Main: /jsonpayload 200
HTTP.Messages.Response:
"""
HTTP/1.1 200 OK
Content-Type: application/json
Transfer-Encoding: chunked
"Hello Adrian""""
```
First, for the two `@show` calls, notice how `jsonpayload` had successfully converted the `POST` data to a `Dict`.
While the `rawpayload` returns the `POST` data as a `String`, exactly as received. Finally, our route handler returns a
JSON response, greeting the user by extracting the name from within the `jsonpayload` `Dict`.
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 1374 | # Handling file uploads
Genie has built-in support for working with file uploads. The collection of uploaded files (as `POST` variables) can be
accessed through the `Requests.filespayload` method. Or, we can retrieve the data corresponding to a given file form
input by using `Requests.filespayload(key)` -- where `key` is the name of the file input in the form.
In the following snippet we configure two routes in the root of the app (`/`): the first route, handling `GET` requests,
displays an upload form. The second route, handling `POST` requests, processes the uploads, generating a file from the
uploaded data, saving it, and displaying the file stats.
---
**HEADS UP**
Notice that we can define multiple routes at the same URL if they have different methods, in our case `GET` and `POST`.
---
### Example
```julia
using Genie, Genie.Router, Genie.Renderer.Html, Genie.Requests
form = """
<form action="/" method="POST" enctype="multipart/form-data">
<input type="file" name="yourfile" /><br/>
<input type="submit" value="Submit" />
</form>
"""
route("/") do
html(form)
end
route("/", method = POST) do
if infilespayload(:yourfile)
write(filespayload(:yourfile))
stat(filename(filespayload(:yourfile)))
else
"No file uploaded"
end
end
up()
```
Upon uploading a file and submitting the form, our app will display the file's stats.
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 2010 | # Adding your existing Julia code into Genie apps
If you have existing Julia code (modules and files) which you'd like to quickly integrate into a web app, Genie provides
an easy way to add and load your code.
## Adding your Julia code to the `lib/` folder
If you have some Julia code which you'd like to integrate in a Genie app, the simplest thing is to add the files to the
`lib/` folder. The files (and folders) in the `lib/` folder are automatically loaded by Genie _recursively_. This means
that you can also add folders under `lib/`, and they will be recursively loaded (included) into the app.
Beware though that this only happens when the Genie app is initially loaded. Hence, an app restart will be required if
you add files and folders after the app is started.
---
**HEADS UP**
Genie won't create the `lib/` folder by default. If the `lib/` folder is not present in the root of the app,
just create it yourself:
```julia
julia> mkdir("lib")
```
---
Once your code is added to the `lib/` folder, it will become available in your app's environment. For example, say we
have a file called `lib/MyLib.jl`:
```julia
# lib/MyLib.jl
module MyLib
using Dates
function isitfriday()
Dates.dayofweek(Dates.now()) == Dates.Friday
end
end
```
Assuming that the name of your Genie app (which is also the name of your main module in `src/`) is `MyGenieApp`, the
modules loaded from `lib/` will be available under the `MyGenieApp` namespace as `MyGenieApp.MyLib`.
---
**HEADS UP**
Instead of using the actual Genie app (main module) name, we can also use the alias `..Main.UserApp`.
---
So we can reference and use our modules in `lib/` in `routes.jl` as follows:
```julia
# routes.jl
using Genie
using MyGenieApp.MyLib # or using ..Main.UserApp.MyLib
route("/friday") do
MyLib.isitfriday() ? "Yes, it's Friday!" : "No, not yet :("
end
```
Use the `lib/` folder to host your Julia code so that Genie knows where to look in order to load it and make it
available throughout the application.
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 4481 | # Deploying Genie apps with Heroku Buildpacks
This tutorial shows how to host a Julia/Genie app using a Heroku Buildpack.
## Prerequisites
This guide assumes you have a Heroku account and are signed into the Heroku CLI.
[Information on how to setup the Heroku CLI is available here](https://devcenter.heroku.com/articles/heroku-cli).
## The application
In order to try the deployment, you will need a sample application. Either pick one of yours or clone this sample one,
as indicated next.
### All Steps (in easy copy-paste format):
Customize your `HEROKU_APP_NAME` to something unique:
```sh
HEROKU_APP_NAME=my-app-name
```
Clone a sample app if needed:
```sh
git clone https://github.com/milesfrain/GenieOnHeroku.git
```
Go into the app's folder:
```sh
cd GenieOnHeroku
```
And create a Heroku app:
```sh
heroku create $HEROKU_APP_NAME --buildpack https://github.com/Optomatica/heroku-buildpack-julia.git
```
Push the newly created app to Heroku:
```sh
git push heroku master
```
Now you can open the app in the browser:
```sh
heroku open -a $HEROKU_APP_NAME
```
If you need to check the logs:
```sh
heroku logs -tail -a $HEROKU_APP_NAME
```
### Steps with more detailed descriptions
#### Select an app name
```sh
HEROKU_APP_NAME=my-app-name
```
This must be unique among all Heroku projects, and is part of the url where your project is hosted (e.g. <https://my-app-name.herokuapp.com/>).
If the name is not unique, you will see this error at the `heroku create` step.
```sh
Creating ⬢ my-app-name... !
▸ Name my-app-name is already taken
```
#### Clone an example project
```sh
git clone https://github.com/milesfrain/GenieOnHeroku.git
cd GenieOnHeroku
```
You may also point to your own project, but it must be a git repo.
A `Procfile` in the root contains the launch command to load your app.
The contents of the `Procfile` for this project is this single line:
```sh
web: julia --project src/app.jl $PORT
```
You may edit the `Procfile` to point to your own project's launch script. (for example `src/my_app_launch_file.jl` instead of `src/app.jl`),
but be sure to take into account the dynamically changing `$PORT` environment variable which is set by Heroku.
If you're deploying a standard Genie application built with `Genie.newapp`, the launch script will be `bin/server`. Genie will automatically pick the `$PORT` number from the environment.
#### Create a Heroku project
```sh
heroku create $HEROKU_APP_NAME --buildpack https://github.com/Optomatica/heroku-buildpack-julia.git
```
This creates a project on the Heroku platform, which includes a separate git repository.
This `heroku` repository is added to the list of tracked repositories and can be observed with `git remote -v`.
```sh
heroku https://git.heroku.com/my-app-name.git (fetch)
heroku https://git.heroku.com/my-app-name.git (push)
origin https://github.com/milesfrain/GenieOnHeroku.git (fetch)
origin https://github.com/milesfrain/GenieOnHeroku.git (push)
```
We are using a buildpack for Julia. This runs many of the common deployment operations required for Julia projects.
It relies on the directory layout found in the example project, with `Project.toml`, `Manifest.toml` in the root,
and all Julia code in the `src` directory.
#### Deploy your app
```sh
git push heroku master
```
This pushes your current branch of your local repo to the `heroku` remote repo's `master` branch.
Heroku will automatically execute the commands described in the Julia buildpack and Procfile of this latest push.
You must push to the heroku `master` branch to trigger an automated deploy.
#### Open your app's webpage
```sh
heroku open -a $HEROKU_APP_NAME
```
This is a convenience command to open your app's webpage in your browser.
The webpage is: `https://$HEROKU_APP_NAME.herokuapp.com/`
For example: <https://my-app-name.herokuapp.com/>
#### View app logs
```sh
heroku logs -tail -a $HEROKU_APP_NAME
```
This is another convenience command to launch a log viewer that remains open to show the latest status of your app.
The `println` statements from Julia will also appear here.
Exit this viewer with `Ctrl-C`.
Logs can also be viewed from the Heroku web dashboard.
For example: <https://dashboard.heroku.com/apps/my-app-name/logs>
### Deploy app updates changes
To deploy any changes made to your app, simply commit those changes locally, and re-push to heroku.
```sh
<make changes>
git commit -am "my commit message"
git push heroku master
```
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 5353 | # Deploying Genie apps to server with Nginx
This tutorial shows how to host a Julia/Genie app on with Nginx.
## Prerequisites
To expose the app over the internet, one needs access to a server. This can be a local machine or a cloud instance such
as AWS EC2 or a Google Cloud Compute Engine for example.
If using a local server, a static IP is needed to ensure continuous access to the app. Internet service provider generally
charge a fee for such extra service.
## The application
We assume that a Genie app has been developed and is ready for deployment and that it is hosted as a project on a git repository.
For example, the app `MyGenieApp` generated through `Genie.Generator.newapp("MyGenieApp")` being hosted at
`github.com/user/MyGenieApp`.
The scripts presented in this tutorial are for Ubuntu 20.04.
## Install and run the Genie app on the server
Access the server:
```sh
ssh -i "ssh-key-for-instance.pem" [email protected]
```
Install Julia if not present. Then make the clone:
```sh
git clone github.com/user/MyGenieApp
cd MyGenieAp
```
Install the app as any other Julia project:
```sh
julia
] activate .
pkg> instantiate
exit()
```
In order to launch the app and exit the console without shutting down the app, we will launch it from a new screen:
```sh
screen -S genie
```
Then set the `GENIE_ENV` environment variable to `prod`:
```sh
export GENIE_ENV=prod
```
Now the application is almost ready to start just need to configure [the secret token](https://genieframework.github.io/Genie.jl/dev/API/secrets.html#Genie.Secrets.secret_token). Go into the project directory and execute the following command. It will generate `secrets.jl` inside `config/secrets.jl` file and if it exists then it will update with a new token string.
```sh
julia --project=. --banner=no --eval="using Pkg; using Genie; Genie.Generator.write_secrets_file()"
```
Launch the app:
```sh
./bin/server
```
Now the Genie app should be running on the server and be accessible at the following address: `123.123.123.123:8000`
(if port 8000 has been open - see instance security settings). Note that you should configure the Genie app so that it
doesn't serve the static content (see the `Settings` option `server_handle_static_file` in `config/env/prod.jl`).
Static content should be handled by nginx. We can now detach from the `genie` screen used to launch the app (Ctl+A d).
## Install and configure nginx server
Nginx server will be used as a reverse proxy. It will listen requests made on port 80 (HTTP) and redirect traffic to the
Genie app running on port 8000 (default Genie setting that can be changed).
Nginx will also be used to serve the app static files, that is, the content under the `./public` folder.
Finally, it can as well handle HTTPS requests, which will also be redirected to the Genie app listening on port 8000.
Installation:
```sh
sudo apt-get update
sudo apt-get install nginx
sudo systemctl start nginx
sudo systemctl enable nginx
```
A configuration file then needs to to be created to indicate on which port to listen (80 for HTTP) and to which port to
redirect the traffic (8000 for default Genie config).
Config is created in folder `/etc/nginx/sites-available`: `sudo nano my-genie-app`.
Put the following content in `my-genie-app`:
```sh
server {
listen 80;
listen [::]:80;
server_name test.com;
root /home/ubuntu/MyGenieApp/public;
index welcome.html;
location / {
proxy_pass http://localhost:8000/;
}
location /css/genie {
proxy_pass http://localhost:8000/;
}
location /img/genie {
proxy_pass http://localhost:8000/;
}
location /js/genie {
proxy_pass http://localhost:8000/;
}
}
```
- `server_name`: refers to the web domain to be used. It can be put to an arbitrary name if the app is only to be served
directly from the server public IP.
- `root`: points to the `public` subfolder where the genie app was cloned.
- `index`: refers to the site index (the landing page).
- The various `location` following the initial proxy to the genie app are used to indicate static content folders to be
served by nginx. These are needed when the `server_handle_static_file` is set to `false` in the Genie app settings.
To make that config effective, it needs to be present in `sites-enabled`. The `default` config can be removed.
```sh
sudo ln -s /etc/nginx/sites-available/my-genie-app /etc/nginx/sites-enabled/my-genie-app
```
Then restart the server to make changes effective:
```sh
sudo systemctl restart nginx
```
## Enable HTTPS
To enable HTTPS, a site-certificate will be needed for the domain on which the site will be served.
A practical approach is to use the utilities provided by [certbot](https://certbot.eff.org/).
Following provided instructions for nginx on Ubuntu 20.04:
```sh
sudo snap install core; sudo snap refresh core
sudo snap install --classic certbot
sudo ln -s /snap/bin/certbot /usr/bin/certbot
```
Then, using certbot utility, a certificate will be generated and appropriate modification to nginx config will be brought to handle support for HTTPS:
```sh
sudo certbot --nginx
```
Note that this step will check for ownernship of the `test.com` domain mentionned in the nginx config file. For that
validation to succeed, it requires to have the `A` record for the domain set to `123.123.123.123`.
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 3690 | # Deploying Genie apps using: A Process Control System
This tutorial shows how to host a Julia/Genie app using process control system. We using `supervisor` for this tutorial.
## Prerequisites
Install `supervisor` in system. If you not sure how to install you can find the suitable command from [here](https://command-not-found.com/supervisord) or you can [refer to official website](http://supervisord.org/)
## The application
We assume that a Genie app has been developed and is ready for deployment and that it is hosted as a project on a git repository.
For example, the app `MyGenieApp` generated through `Genie.Generator.newapp("MyGenieApp")` being hosted at
`github.com/user/MyGenieApp`.
The scripts presented in this tutorial are for Ubuntu 20.04.
## Install and run the Genie app on the server
Access the server:
```shell
ssh -i "ssh-key-for-instance.pem" [email protected]
```
Install Julia if not present. Then make the clone:
```shell
git clone github.com/user/MyGenieApp
cd MyGenieAp
```
Install the app as any other Julia project:
```shell
julia
] activate .
pkg> instantiate
exit()
```
In order to launch the app using `supervisor` you have to create `genie-supervisor.conf` file or you can name it something else with file name ending `.conf` as extension at project directory path.
```shell
[program:genie-application]
process_name=%(program_name)s_%(process_num)02d
command= ./bin/server
autostart=true
autorestart=true
stopasgroup=true
killasgroup=true
numprocs=1
redirect_stderr=true
stdout_logfile=/var/log/genie-application.log
stopwaitsecs=3600
```
Now the application is almost ready to start just need to configure [the secret token](https://genieframework.github.io/Genie.jl/dev/API/secrets.html#Genie.Secrets.secret_token). Go into the project directory and execute the following command. It will generate `secrets.jl` inside `config/secrets.jl` file and if it exists then it will update with a new token string.
```sh
julia --project=. --banner=no --eval="using Pkg; using Genie; Genie.Generator.write_secrets_file()"
```
Then set the `GENIE_ENV` environment variable to `prod`:
```shell
export GENIE_ENV=prod
```
To launch the app few things need to do before starting process control.
Create symbolic link to supervisor config directory
```shell
cd /etc/supervisor/conf.d/
sudo ln -s /PATH TO THE SUPERVISOR.CONF FILE
sudo /etc/init.d/supervisor reload
```
### Few assumption about installation instruction
- In this tutorial above configuration is done at `Debian` or `Ubutu` server and tested well
- `GENIE_ENV=prod` should be exported before you run the application else it will take default `GENIE_ENV` environment
### If thing not working then you may check following things.
(1) check the `sudo systemctl status supervisor.service` service is working or not. if not working then you can start and enable for the start up using following command
```shell
sudo systemctl enable supervisor.service
sudo systemctl start supervisor.service
```
and then check if status is active or still has any issue.
(2) You can check the application log by tail to log file as bellow
```shell
tail -f /var/log/genie-application.log
```
in this log you will find the genie logs.
(3) Make sure whatever port you used inside `GENIE_ENV` config file at `server_port` must be open in at firewall and no other process is bind to it.
Check weather the any process is running at port 80 for an example use following command
```shell
sudo lsof -t -i:80
```
If you want to kill the process which is running at port 80 you can use single liner magic command
```shell
if sudo lsof -t -i:80; then sudo kill -9 $(sudo lsof -t -i:80); fi
``` | Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 131 | ---
numbers: [1, 1, 2, 3, 5, 8, 13]
---
# There are $(length(numbers))
$(
for_each(numbers) do number
" -> $number"
end
) | Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"MIT"
]
| 5.30.6 | 376aa3d800f4da32d232f2d9a1685e34e2911b4a | docs | 91 | # There are $(length(numbers))
$(
for_each(numbers) do number
" -> $number"
end
)
| Genie | https://github.com/GenieFramework/Genie.jl.git |
|
[
"BSD-3-Clause"
]
| 0.1.2 | 7cade784ab71f8a594597382852143e5f25af0e7 | code | 343 | push!(LOAD_PATH,"../src/")
using Documenter
using HumanReadableSExpressions
makedocs(
sitename = "HumanReadableSExpressions.jl",
format = Documenter.HTML(),
modules = [HumanReadableSExpressions]
)
deploydocs(
repo = "github.com/lukebemish/HumanReadableSExpressions.jl.git",
target = "build",
push_preview = true
)
| HumanReadableSExpressions | https://github.com/lukebemish/HumanReadableSExpressions.jl.git |
|
[
"BSD-3-Clause"
]
| 0.1.2 | 7cade784ab71f8a594597382852143e5f25af0e7 | code | 8075 | module HumanReadableSExpressions
export HrseReadOptions, HrsePrintOptions, readhrse, writehrse, ashrse
import StructTypes
"""
An extension to HRSE's syntax that affects parsing and/or printing.
See also [`DENSE`](@ref).
"""
@enum Extension DENSE=1
"""
An extension to HRSE; axpects a "dense" HRSE file with a single root-level element instead of a list of elements.
See also [`Extension`](@ref).
"""
DENSE;
"""
HrseReadOptions(kwargs...)
Stores options for parsing HRSE files.
# Arguments
- `integertypes = [Int64, BigInt]`: A list of signed integer types to try parsing integers as. The first type that can
represent the integer will be used.
- `floattype = Float64`: The floating point type to parse floating point numbers as.
- `readcomments = false`: Whether to read comments and store them in `CommentedElement` objects; if false, comments are
ignored.
- `extensions`: A collection of [`Extension`](@ref)s to HRSE.
See also [`readhrse`](@ref).
"""
struct HrseReadOptions
integertypes
floattype::Type{<:AbstractFloat}
readcomments::Bool
extensions
HrseReadOptions(;
integertypes = [Int64, BigInt],
floattype::Type{<:AbstractFloat} = Float64,
readcomments::Bool = false,
extensions=[]) = new(
integertypes,
floattype,
readcomments,
extensions)
end
"""
A flag that decides how pairs are displayed while printing a HRSE structure.
See also [`CONDENSED_MODE`](@ref), [`DOT_MODE`](@ref), [`EQUALS_MODE`](@ref), [`COLON_MODE`](@ref).
"""
@enum PairMode CONDENSED_MODE=1 DOT_MODE=2 EQUALS_MODE=3 COLON_MODE=4
"""
A pair printing mode; pairs should all be condensed to a single line using classic s-expressions, eleminating as many spaces
as possible.
See also [`PairMode`](@ref).
"""
CONDENSED_MODE;
"""
A pair printing mode; pairs should all be displayed using classic s-expressions with dot-deliminated pairs, using
indentation to show nesting.
See also [`PairMode`](@ref).
"""
DOT_MODE;
"""
A pair printing mode; pairs should all be displayed using equals-sign-deliminated pairs with implied parentheses, using
indentation to show nesting.
See also [`PairMode`](@ref).
"""
EQUALS_MODE;
"""
A pair printing mode; pairs should all be displayed using colon-deliminated pairs with implied parentheses, using
indentation to show nesting and avoiding parentheses around lists as possible.
See also [`PairMode`](@ref).
"""
COLON_MODE;
"""
HrsePrintOptions(kwargs...)
Stores options for printing HRSE structures to text.
# Arguments
- `indent = " "`: The string to use for indentation.
- `comments = true`: Whether to print comments from `CommentedElement` objects; if false, comments are ignored.
- `extensions`: A collection of [`Extension`](@ref)s to HRSE.
- `pairmode = COLON_MODE`: The [`PairMode`](@ref) to use when printing pairs.
- `inlineprimitives = 20`: The maximum string length of a list of primitives to print on a single line instead of adding
a new indentation level.
- `trailingnewline = true`: Whether to print a trailing newline at the end of the file.
See also [`writehrse`](@ref), [`ashrse`](@ref).
"""
struct HrsePrintOptions
indent::String
comments::Bool
extensions
pairmode::PairMode
inlineprimitives::Integer
trailingnewline::Bool
HrsePrintOptions(;
indent::String=" ",
comments::Bool=true,
extensions=[],
trailingnewline::Bool=true,
pairmode::PairMode=COLON_MODE,
inlineprimitives::Integer=20) = new(
indent,
comments,
extensions,
pairmode,
inlineprimitives,
trailingnewline)
end
"""
CommentedElement(element, comments)
Wraps an element with a list of comments which directly precede it attached to it.
"""
struct CommentedElement
element::Any
comments::Vector{String}
end
include("literals.jl")
include("parser.jl")
include("structures.jl")
include("printer.jl")
"""
readhrse(hrse::IO; options=HrseReadOptions(); type=nothing)
readhrse(hrse::String; options=HrseReadOptions(); type=nothing)
Reads an HRSE file from the given IO object or string and returns the corresponding Julia object. The `options` argument can
be used to configure the parser. Lists will be read as vectors, pairs as a Pair, symbols and strings as a String, and
numeric types as the corresponding Julia type defined in the parser options. If `type` is given, the result will be parsed
as the given type using its StructTypes.StructType.
# Examples
```jldoctest
julia> using HumanReadableSExpressions
julia> hrse = \"\"\"
alpha:
1 2 3 4
5 6
7 8 9
beta: (0 . 3)
gamma:
a: 1
b: 2
c: "c"
\"\"\";
julia> readhrse(hrse)
3-element Vector{Pair{String}}:
"alpha" => [[1, 2, 3, 4], [5, 6], [7, 8, 9]]
"beta" => (0 => 3)
"gamma" => Pair{String}["a" => 1, "b" => 2, "c" => "c"]
```
See also [`HrseReadOptions`](@ref).
"""
function readhrse(hrse::IO; options::HrseReadOptions=HrseReadOptions(), type::Union{Type, Nothing}=nothing)
dense = DENSE in options.extensions
source = Parser.LexerSource(hrse, options)
Parser.runmachine(source)
tokens = Parser.Tokens(source.tokens, nothing, [])
parsetree = Parser.parsefile(tokens, options)
# discard trailing comments
Parser.parsecomments(tokens, options)
Parser.stripindent(tokens)
if Parser.tokentype(Parser.peek(tokens)) != Parser.EOF
throw(Parser.HrseSyntaxException("Unexpected token '$(Parser.tokentext(Parser.peek(tokens)))'", Parser.tokenline(Parser.peek(tokens)), Parser.tokenpos(Parser.peek(tokens))))
end
translated = Parser.translate(parsetree, options)
if (dense && length(translated) != 1)
throw(Parser.HrseSyntaxException("Expected a single root-level element in dense mode", Parser.tokenline(Parser.peek(tokens)), Parser.tokenpos(Parser.peek(tokens))))
end
obj = dense ? translated[1] : translated
if type !== nothing
return Structures.deserialize(StructTypes.StructType(type), obj, type, options)
end
return obj
end
readhrse(hrse::String; options::HrseReadOptions=HrseReadOptions(), type::Union{Type, Nothing}=nothing) = readhrse(IOBuffer(hrse), options=options, type=type)
"""
writehrse(io::IO, obj, options::HrsePrintOptions)
writehrse(obj, options::HrsePrintOptions)
Writes the given Julia object to the given IO object as a HRSE file. The `options` argument can be used to configure the
behavior of the printer. If no IO object is given, the output is written to `stdout`. Arbitrary objects are serialized
using their StructTypes.StructType.
# Examples
```jldoctest
julia> using HumanReadableSExpressions
julia> hrse = [
:alpha => [
[1, 2, 3, 4],
[5, 6],
[7, 8, 9]
],
:beta => (0 => 3),
:gamma => [
:a => 1
:b => 2
:c => :c
]
];
julia> writehrse(hrse, HumanReadableSExpressions.HrsePrintOptions())
alpha:
1 2 3 4
5 6
7 8 9
beta: 0: 3
gamma:
a: 1
b: 2
c: c
```
See also [`HrsePrintOptions`](@ref).
"""
function writehrse(io::IO, obj, options::HrsePrintOptions)
toprint = (DENSE in options.extensions) ? [obj] : obj
if options.pairmode == CONDENSED_MODE
o = Printer.translate(toprint, options)
for i in o Printer.condensed(io, i, options) end
else
Printer.pretty(io, toprint, options, 0, true; root=true)
if options.trailingnewline
println(io)
end
end
end
writehrse(obj, options::HrsePrintOptions) = writehrse(stdout, obj, options)
"""
ashrse(obj, options::HrsePrintOptions)
Returns the given Julia object as a string containing a HRSE file. The `options` argument can be used to configure the
behavior of the printer.
See also [`writehrse`](@ref), [`HrsePrintOptions`](@ref).
"""
ashrse(obj, options::HrsePrintOptions) = begin
io = IOBuffer()
writehrse(io, obj, options)
return String(take!(io))
end
end # module Hrse
| HumanReadableSExpressions | https://github.com/lukebemish/HumanReadableSExpressions.jl.git |
|
[
"BSD-3-Clause"
]
| 0.1.2 | 7cade784ab71f8a594597382852143e5f25af0e7 | code | 4544 | module Literals
import Base.Checked: add_with_overflow, mul_with_overflow
const BASE10::UInt16 = 10
const BASE16::UInt16 = 16
const BASE2::UInt16 = 2
#=
( and ) reserved for s-expressions
:, ., = reserved for pairs
" reserved for strings
; reserved for comments
# reserved for literals
whitespace can't be in symbols in general
', ` reserved for extensions
=#
function category_code(c::AbstractChar)
!Base.Unicode.ismalformed(c) ? category_code(UInt32(c)) : Cint(31)
end
function category_code(x::Integer)
x ≤ 0x10ffff ? ccall(:utf8proc_category, Cint, (UInt32,), x) : Cint(30)
end
const CATEGORY_PC = 12
const CATEGORY_PD = 13
const CATEGORY_CF = 27
function isfmt(char::Char)
category_code(char) == CATEGORY_CF
end
function issymbolstartbanned(char::Char)
return isspace(char) ||
iscntrl(char) || isfmt(char) ||
char in ['(', ')', '+', '-', '"', '\'', '`', ':', ';', '#', '.', '='] ||
(!isascii(char) && ispunct(char)) ||
isnumeric(char)
end
function issymbolstart(char::Char)
return !issymbolstartbanned(char)
end
function issymbolbody(char::Char)
return char in ['+', '-'] || category_code(char) == CATEGORY_PC || category_code(char) == CATEGORY_PD || isnumeric(char) || issymbolstart(char)
end
function issymbol(str)
return !isempty(str) && issymbolstart(str[1]) && all(issymbolbody, str[2:end])
end
const DEC_DIGIT = "0-9"
const HEX_DIGIT = "0-9a-fA-F"
const BIN_DIGIT = "0-1"
const INT_REGEX = Regex("^([+-]?)((0[xX][$(HEX_DIGIT)_]*[$(HEX_DIGIT)][$(HEX_DIGIT)_]*)|(0[bB][$(BIN_DIGIT)_]*[$(BIN_DIGIT)][$(BIN_DIGIT)_]*)|([$(DEC_DIGIT)_]*[$(DEC_DIGIT)][$(DEC_DIGIT)_]*))\$")
const DEC_LITERAL = "([$(DEC_DIGIT)_]*[$(DEC_DIGIT)][$(DEC_DIGIT)_]*)"
const FLOAT_EXPONENT = "[eE][+-]?$DEC_LITERAL"
const FLOAT_MAIN = "($DEC_LITERAL\\.$DEC_LITERAL?|\\.$DEC_LITERAL)"
const FLOAT_REGEX = Regex("^[+-]?($FLOAT_MAIN($FLOAT_EXPONENT)?|($DEC_LITERAL$FLOAT_EXPONENT))\$")
function parseint(s::String; types=[Int64, BigInt])
types = Vector{Type{<:Signed}}(types)
s = s*" "
s = replace(s, '_'=>"")
if startswith(s, '-')
return -parseunsigned(s[2:end], types)
elseif startswith(s, '+')
return parseunsigned(s[2:end], types)
end
return parseunsigned(s, types)
end
function parsefloat(s::String; type::Type{<:AbstractFloat}=Float64)
return tryparse(type, s)
end
function parseunsigned(s::String, types::Vector{Type{<:Signed}})
if startswith(s, "0x")
return parseint(s[3:end], BASE16, types[1], types[2:end], zero(types[1]))
elseif startswith(s, "0b")
return parseint(s[3:end], BASE2, types[1], types[2:end], zero(types[1]))
end
return parseint(s, BASE10, types[1], types[2:end], zero(types[1]))
end
function parseint(s::String, base::UInt16, ::Type{BigInt}, ::Vector{Type{<:Signed}}, n::BigInt)::Union{BigInt, Nothing}
i = 0
base = convert(BigInt, base)
endpos = length(s)
c, i = iterate(s,1)::Tuple{Char, Int}
while !isspace(c)
d = convert(BigInt, parsecharint(c))
n *= base
n += d
c, i = iterate(s,i)::Tuple{Char, Int}
i > endpos && break
end
return n
end
function parseint(s::String, base::UInt16, ::Type{T}, overflowtypes::Vector{Type{<:Signed}}, n::T)::Union{Integer, Nothing} where T <: Signed
i = 0
m::T = if base == BASE10
div(typemax(T) - T(9), T(10))
elseif base == BASE16
div(typemax(T) - T(15), T(16))
elseif base == BASE2
div(typemax(T) - T(1), T(2))
end
baseT = convert(T, base)
endpos = length(s)
c, i = iterate(s,1)::Tuple{Char, Int}
while n <= m
d = convert(T, parsecharint(c))
n *= baseT
n += d
c, i = iterate(s,i)::Tuple{Char, Int}
i >= endpos && break
end
while !isspace(c)
nold = n
d = convert(T, parsecharint(c))
n, ov_mul = mul_with_overflow(n, baseT)
n, ov_add = add_with_overflow(n, d)
if ov_mul || ov_add
if length(overflowtypes) == 0
return nothing
else
newtype = overflowtypes[1]
return parseint(s[i-1:end], base, newtype, overflowtypes[2:end], convert(newtype, nold))
end
end
c, i = iterate(s,i)::Tuple{Char, Int}
i > endpos && break
end
return n
end
function parsecharint(c::Char)::UInt32
i = reinterpret(UInt32, c)>>24
if i < 0x40
return i - 0x30
end
return i - 0x57
end
end | HumanReadableSExpressions | https://github.com/lukebemish/HumanReadableSExpressions.jl.git |
|
[
"BSD-3-Clause"
]
| 0.1.2 | 7cade784ab71f8a594597382852143e5f25af0e7 | code | 26164 | module Parser
import ..HumanReadableSExpressions
import ..Literals
import ..Literals: issymbolstartbanned, issymbolstart, issymbolbody
import Base: eof, push!
import Unicode
abstract type Token end
struct SimpleToken <: Token
type::Symbol
line::Integer
pos::Integer
end
struct StringToken <: Token
value::String
line::Integer
pos::Integer
multiline::Bool
lastline::Bool
StringToken(value::String, line::Integer, pos::Integer, multiline::Bool; lastline::Bool=false) = new(value, line, pos, multiline, lastline)
end
struct NumberToken <: Token
value::Number
line::Integer
pos::Integer
end
struct CommentToken <: Token
comment::String
line::Integer
pos::Integer
end
struct IndentToken <: Token
indent::String
line::Integer
pos::Integer
end
tokentext(t::SimpleToken) = t.type
tokentext(t::StringToken) = t.value
tokentext(t::NumberToken) = t.value
tokentext(t::CommentToken) = t.comment
tokentext(t::IndentToken) = t.indent
tokenline(t::SimpleToken) = t.line
tokenline(t::StringToken) = t.line
tokenline(t::NumberToken) = t.line
tokenline(t::CommentToken) = t.line
tokenline(t::IndentToken) = t.line
tokenpos(t::SimpleToken) = t.pos
tokenpos(t::StringToken) = t.pos
tokenpos(t::NumberToken) = t.pos
tokenpos(t::CommentToken) = t.pos
tokenpos(t::IndentToken) = t.pos
const STRING = :STRING
const NUMBER = :NUMBER
const COMMENT = :COMMENT
tokentype(t::SimpleToken) = t.type
tokentype(::StringToken) = STRING
tokentype(::NumberToken) = NUMBER
tokentype(::CommentToken) = COMMENT
tokentype(::IndentToken) = INDENT
tokentype(::Nothing) = nothing
const LPAREN = :LPAREN
const RPAREN = :RPAREN
const EQUALS = :EQUALS
const DOT = :DOT
const COLON = :COLON
const INDENT = :INDENT
const EOF = :EOF
const TRUE = :TRUE
const FALSE = :FALSE
const S_MODE = :S_MODE
const I_MODE = :I_MODE
struct HrseSyntaxException <: Exception
msg::String
line::Integer
pos::Integer
end
Base.showerror(io::IO, e::HrseSyntaxException) = begin
print(io, "HrseSyntaxException: ", e.msg, " at line ", e.line, ", column ", e.pos)
end
struct Position
line::Integer
pos::Integer
end
mutable struct LexerSource
leading::Vector{Char}
positions::Vector{Position}
io::IO
line::Integer
pos::Integer
tokens::Vector{Token}
options::HumanReadableSExpressions.HrseReadOptions
LexerSource(io::IO, options::HumanReadableSExpressions.HrseReadOptions) = new(Char[], Position[], io, 1, 0, Token[], options)
end
function emit(source::LexerSource, token::Token)
if (tokentype(token) == INDENT || tokentype(token) == EOF) &&
length(source.tokens) > 0 && tokentype(source.tokens[end]) == INDENT
source.tokens[end] = token
return source.tokens
end
push!(source.tokens, token)
end
function consume(source::LexerSource)
if length(source.leading) == 0 && eof(source.io)
return nothing
end
if length(source.leading) == 0
c = read(source.io, Char)
if c == '\n'
source.line += 1
source.pos = 0
else
source.pos += 1
end
return c
else
c = popfirst!(source.leading)
pos = popfirst!(source.positions)
source.line = pos.line
source.pos = pos.pos
return c
end
end
function peek(source::LexerSource; num=1)
while length(source.leading) < num
if eof(source.io)
return nothing
end
c = read(source.io, Char)
lastpos = length(source.leading) == 0 ? Position(source.line, source.pos) : source.positions[end]
push!(source.leading, c)
pos = lastpos.pos
line = lastpos.line
if c == '\n'
line += 1
pos = 0
else
pos += 1
end
push!(source.positions, Position(line, pos))
end
return source.leading[num]
end
function peekpos(source::LexerSource; num=1)
peek(source, num=num)
return length(source.leading) >= num ? source.positions[num] : source.positions[end]
end
function iswhitespace(char::Char)
return char == ' ' || char == '\t'
end
function isnumericbody(char::Char)
return isxdigit(char) || char == '_'
end
function runmachine(source::LexerSource)
machine = source -> lexindent(source, Char[], 1, 1)
while machine !== nothing
machine = machine(source)
end
end
function lex(source::LexerSource)
char = consume(source)
line = source.line
pos = source.pos
if char === nothing
emit(source, SimpleToken(EOF, source.line, source.pos))
return
elseif char == '"'
multiline = peek(source) == '"' && peek(source, num=2) == '"'
if multiline
consume(source)
consume(source)
end
return s -> lexstring(s, Char[], line, pos, multiline)
elseif char == '('
return s -> lexparen(s)
elseif char == ')'
emit(source, SimpleToken(RPAREN, source.line, source.pos))
elseif char == '='
emit(source, SimpleToken(EQUALS, source.line, source.pos))
elseif char == '.'
return s -> lexdot(s)
elseif char == ':'
emit(source, SimpleToken(COLON, source.line, source.pos))
elseif char == ';'
return s -> lexcomment(s, Char[], line, pos)
elseif iswhitespace(char)
# just continue
elseif issymbolstart(char)
return s -> lexsymbol(s, [char])
elseif isnumericbody(char) || char in ['.', '+', '-']
return s -> lexnumber(s, [char], line, pos)
elseif char == '#'
return s -> lexliteral(s, Char['#'], line, pos)
elseif char == '\n'
return s -> lexindent(s, Char[], line, pos+1)
else
throw(HrseSyntaxException("Unexpected character '$char'", source.line, source.pos))
end
return s -> lex(s)
end
function lexindent(source, chars, line, pos)
next = peek(source)
if next == ' ' || next == '\t'
push!(chars, consume(source))
return s -> lexindent(s, chars, line, pos)
else
emit(source, IndentToken(String(chars), line, pos))
return s -> lex(s)
end
end
function lexparen(source::LexerSource)
next = peek(source)
line = source.line
pos = source.pos
if next == ';'
return s -> lexlistcommentopen(s, 0, line, pos)
end
emit(source, SimpleToken(LPAREN, source.line, source.pos))
return s -> lex(s)
end
function lexcomment(source::LexerSource, chars, line, pos)
char = peek(source)
if char === nothing || char == '\n'
comment = strip(String(chars))
emit(source, CommentToken(comment, line, pos))
return s -> lex(s)
else
push!(chars, consume(source))
return s -> lexcomment(s, chars, line, pos)
end
end
function lexlistcommentopen(source::LexerSource, count, line, pos)
char = peek(source)
if char === nothing
throw(HrseSyntaxException("Unterminated multiline comment", line, pos))
elseif char == ';'
consume(source)
return s -> lexlistcommentopen(s, count+1, line, pos)
else
return s -> lexlistcommentbody(s, Char[], count, 0, line, pos)
end
end
function lexlistcommentbody(source::LexerSource, chars, count, counting, line, pos)
char = consume(source)
if char === nothing
throw(HrseSyntaxException("Unterminated multiline comment", line, pos))
elseif char == ')'
if counting == count
comment = strip(String(chars))
emit(source, CommentToken(comment, line, pos))
return s -> lex(s)
else
if counting != 0
for _ in 1:counting
push!(chars, ';')
end
end
push!(chars, char)
return s -> lexlistcommentbody(s, chars, count, 0, line, pos)
end
elseif char == ';'
return s -> lexlistcommentbody(s, chars, count, counting+1, line, pos)
else
if counting != 0
for _ in 1:counting
push!(chars, ';')
end
end
push!(chars, char)
return s -> lexlistcommentbody(s, chars, count, 0, line, pos)
end
end
function lexnumber(source::LexerSource, chars, line::Integer, pos::Integer)
char = peek(source)
if char !== nothing && (isnumericbody(char) || char in ['.', 'e', 'E', '+', '-', 'x', 'X', 'b', 'B'])
push!(chars, consume(source))
return s -> lexnumber(s, chars, line, pos)
elseif char == '#' && length(chars) == 1
return s -> lexliteral(s, Char[chars[1], consume(source)], line, pos)
else
next = peek(source)
if !isnothing(next) && issymbolbody(next)
throw(HrseSyntaxException("Invalid number '$(String(chars))$(next)'", line, pos))
end
text = String(chars)
if !isnothing(match(Literals.FLOAT_REGEX, text))
parsed = Literals.parsefloat(replace(text, '_'=>""); type=source.options.floattype)
if parsed === nothing
throw(HrseSyntaxException("Invalid number '$text'", line, pos))
end
emit(source, NumberToken(parsed, line, pos))
elseif !isnothing(match(Literals.INT_REGEX, text))
parsed = Literals.parseint(text; types=source.options.integertypes)
if parsed === nothing
throw(HrseSyntaxException("Invalid number '$text'", line, pos))
end
emit(source, NumberToken(parsed, line, pos))
else
throw(HrseSyntaxException("Invalid number '$text'", line, pos))
end
return s -> lex(s)
end
end
function lexliteral(source::LexerSource, chars, line::Integer, pos::Integer)
char = peek(source)
if char === nothing || !issymbolbody(char)
text = String(chars)
if text == "#t"
emit(source, SimpleToken(TRUE, line, pos))
elseif text == "#f"
emit(source, SimpleToken(FALSE, line, pos))
elseif text == "#inf" || text == "+#inf"
emit(source, NumberToken(source.options.floattype(Inf), line, pos))
elseif text == "-#inf"
emit(source, NumberToken(source.options.floattype(-Inf), line, pos))
elseif text == "#nan"
emit(source, NumberToken(source.options.floattype(NaN), line, pos))
else
throw(HrseSyntaxException("Invalid literal '$text'", line, pos))
end
return s -> lex(s)
else
push!(chars, consume(source))
return s -> lexliteral(s, chars, line, pos)
end
end
function lexdot(source::LexerSource)
i = 1
digits = false
while (char = peek(source, num=i)) !== nothing && isdigit(char) && isnumericbody(char)
i += 1
if isdigit(char)
digits = true
end
end
if digits
line = source.line
pos = source.pos
return s -> lexnumber(s, Char['.'], line, pos)
else
emit(source, SimpleToken(DOT, source.line, source.pos))
end
return s -> lex(s)
end
function lexsymbol(source::LexerSource, chars)
char = peek(source)
if char === nothing || !issymbolbody(char)
emit(source, StringToken(String(chars), source.line, source.pos, false))
return s -> lex(s)
else
push!(chars, consume(source))
return s -> lexsymbol(s, chars)
end
end
function lexstring(source::LexerSource, chars, startline::Integer, startpos::Integer, multiline::Bool)
char = consume(source)
if char === nothing
throw(HrseSyntaxException("Unterminated string", startline, startpos))
elseif char == '\\'
return s -> lexescape(s, chars, startline, startpos, multiline)
elseif char == '\n' && !multiline
throw(HrseSyntaxException("Unterminated string", startline, startpos))
elseif char == '\n' && multiline
emit(source, StringToken(String(chars), startline, startpos, multiline))
empty!(chars)
elseif iscntrl(char) && char != '\t' && char != '\r' && char != '\n'
throw(HrseSyntaxException("Invalid control character in string: U+$(string(UInt16(char), base=16, pad=4))", startline, startpos))
elseif char == '"'
if multiline && (peek(source) != '"' || peek(source, num=2) != '"')
# Just continue
else
if multiline
consume(source)
consume(source)
end
next = peek(source)
if next === nothing
# pass
elseif issymbolbody(next)
pos = peekpos(source)
throw(HrseSyntaxException("Unexpected symbol character directly after string", pos.line, pos.pos))
elseif next == '"'
pos = peekpos(source)
throw(HrseSyntaxException("Unexpected double quote directly after string", pos.line, pos.pos))
end
emit(source, StringToken(String(chars), startline, startpos, multiline; lastline = true))
return s -> lex(s)
end
end
if (char != '\r' && char != '\n')
push!(chars, char)
end
return s -> lexstring(s, chars, startline, startpos, multiline)
end
function lexescape(source::LexerSource, chars, startline::Integer, startpos::Integer, multiline::Bool)
char = consume(source)
callback = (s, c) -> lexstring(s, push!(chars, c), startline, startpos, multiline)
if char === nothing
throw(HrseSyntaxException("Unterminated string", startline, startpos))
elseif char == 'n'
push!(chars, '\n')
elseif char == 't'
push!(chars, '\t')
elseif char == 'r'
push!(chars, '\r')
elseif char == 'b'
push!(chars, '\b')
elseif char == 'f'
push!(chars, '\f')
elseif char == 'v'
push!(chars, '\v')
elseif char == 'a'
push!(chars, '\a')
elseif char == 'e'
push!(chars, '\e')
elseif char == '\\'
push!(chars, '\\')
elseif char == '"'
push!(chars, '"')
elseif multiline && char == '\n'
return s -> lexwhitespace(s, chars, startline, startpos, multiline)
elseif char == 'u'
return s -> lexunicode(s, callback)
elseif '0' <= char < '8'
return s -> lexoctal(s, callback, [char])
else
throw(HrseSyntaxException("Invalid escape sequence '\\$(char)'", source.line, source.pos))
end
return s -> lexstring(s, chars, startline, startpos, multiline)
end
function lexwhitespace(source::LexerSource, chars, startline::Integer, startpos::Integer, multiline::Bool)
char = peek(source)
if char === nothing
throw(HrseSyntaxException("Unterminated string", startline, startpos))
elseif iscntrl(char) && char != '\t' && char != '\r' && char != '\n'
throw(HrseSyntaxException("Invalid control character in string: U+$(string(UInt16(char), base=16, pad=4))", startline, startpos))
elseif char == '\n' || isspace(char)
consume(source)
return s -> lexwhitespace(s, chars, startline, startpos, multiline)
end
return s -> lexstring(s, chars, startline, startpos, multiline)
end
function lexunicode(source::LexerSource, callback)
char = consume(source)
if char == '{'
return s -> lexunicodepoint(s, Char[], callback)
else
throw(HrseSyntaxException("Invalid unicode character escape \"\\u$char\"", source.line, source.pos))
end
end
function lexoctal(source::LexerSource, callback, chars)
next = peek(source)
if length(chars) < 3 && '0' <= next < '8'
char = consume(source)
push!(chars, char)
return s -> lexoctal(s, callback, chars)
else
i = tryparse(UInt8, String(chars); base=8)
if i === nothing
throw(HrseSyntaxException("Invalid octal character \"\\$(String(chars))\"", source.line, source.pos))
end
return s -> callback(s, i)
end
end
function lexunicodepoint(source::LexerSource, chars, callback)
char = consume(source)
if char == '}'
i = tryparse(UInt32, String(chars); base=16)
if i === nothing || !Unicode.isassigned(i)
throw(HrseSyntaxException("Invalid unicode character \"\\u{$(String(chars))}\"", source.line, source.pos))
end
u = Char(i)
return s -> callback(s, i)
elseif isxdigit(char)
push!(chars, char)
return s -> lexunicodepoint(s, chars, callback)
else
throw(HrseSyntaxException("Invalid unicode character escape \"\\u{$(String(chars))}$char\"", source.line, source.pos))
end
end
mutable struct Tokens
tokens
indentlevel
rootlevel
end
peek(tokens::Tokens) = tokens.tokens[1]
peek(tokens::Tokens, i::Integer) = length(tokens.tokens) >= i ? tokens.tokens[i] : nothing
push!(tokens::Tokens, token::Token) = pushfirst!(tokens.tokens, token)
function consume(tokens::Tokens)
token = popfirst!(tokens.tokens)
if tokentype(token) == INDENT
tokens.indentlevel = token
end
return token
end
abstract type Expression end
struct DotExpression <: Expression
left::Expression
right::Expression
end
struct ListExpression <: Expression
expressions::Vector{Expression}
end
struct StringExpression <: Expression
string::String
end
struct BoolExpression <: Expression
value::Bool
end
struct NumberExpression <: Expression
value::Number
end
struct CommentExpression <: Expression
comments::Vector{String}
expression::Expression
end
function parsefile(tokens, options::HumanReadableSExpressions.HrseReadOptions)
inner = Expression[]
baseindent = peek(tokens)
comments = []
if tokentype(baseindent) == INDENT
if isempty(tokens.rootlevel) || startswith(baseindent.indent, tokens.rootlevel[end].indent)
while tokentype(peek(tokens)) != EOF && tokentype(peek(tokens)) != RPAREN
comments = parsecomments(tokens, options)
peeked = peek(tokens)
if tokentype(peeked) == INDENT
following = tokentype(peek(tokens, 2))
if following == EOF || following == RPAREN
consume(tokens)
break
end
if peeked.indent == baseindent.indent
elseif peeked.indent != tokens.rootlevel[end].indent
throw(HrseSyntaxException("Unexpected indent level", peeked.line, peeked.pos))
else
break
end
end
expression = parseexpression(tokens, options)
if !isempty(comments)
expression = CommentExpression([i.comment for i in comments], expression)
comments = []
end
push!(inner, expression)
end
if !isempty(comments)
for comment in reverse(comments)
push!(tokens, comment)
end
end
if !isempty(tokens.rootlevel) pop!(tokens.rootlevel) end
return ListExpression(inner)
end
end
throw(HrseSyntaxException("Expected indent", peek(tokens).line, peek(tokens).pos))
end
function stripindent(tokens)
indent = peek(tokens)
if tokentype(indent) == INDENT
return consume(tokens)
end
end
function parsecomments(tokens, options::HumanReadableSExpressions.HrseReadOptions)
comments = []
indent = stripindent(tokens)
while tokentype(peek(tokens)) == COMMENT
push!(comments, consume(tokens))
newindent = stripindent(tokens)
if !isnothing(newindent)
indent = newindent
end
end
if !isnothing(indent)
push!(tokens, indent)
end
return options.readcomments ? comments : []
end
function parseexpression(tokens, options::HumanReadableSExpressions.HrseReadOptions)
comments = parsecomments(tokens, options)
if !isempty(comments)
return CommentExpression([i.comment for i in comments], parseexpression(tokens, options))
end
expression = parsecompleteexpression(tokens, options)
if tokentype(peek(tokens)) == COLON
consume(tokens)
if tokentype(peek(tokens)) == INDENT
push!(tokens.rootlevel, tokens.indentlevel)
comments = parsecomments(tokens, options)
indent = peek(tokens)
for comment in reverse(comments)
push!(tokens, comment)
end
if startswith(tokens.rootlevel[end].indent, indent.indent)
pop!(tokens.rootlevel)
return DotExpression(expression, ListExpression([]))
elseif startswith(indent.indent, tokens.indentlevel.indent)
return DotExpression(expression, parsefile(tokens, options))
else
throw(HrseSyntaxException("Unexpected indent level", indent.line, indent.pos))
end
else
return DotExpression(expression, parseexpression(tokens, options))
end
elseif tokentype(peek(tokens)) == EQUALS
consume(tokens)
return DotExpression(expression, parseexpression(tokens, options))
else
return expression
end
end
function parsecompleteexpression(tokens, options::HumanReadableSExpressions.HrseReadOptions)
if tokentype(peek(tokens)) == LPAREN
return parselistexpression(tokens, options)
elseif tokentype(peek(tokens)) == STRING
return parsestringexpression(tokens, options)
elseif tokentype(peek(tokens)) == TRUE || tokentype(peek(tokens)) == FALSE
return parseboolexpression(tokens, options)
elseif tokentype(peek(tokens)) == NUMBER
token = consume(tokens)
return NumberExpression(token.value)
elseif tokentype(peek(tokens)) == INDENT
return parseimodelineexpression(tokens, options)
else
throw(HrseSyntaxException("Unexpected token '$(tokentext(peek(tokens)))'", tokenline(peek(tokens)), tokenpos(peek(tokens))))
end
end
function parselistexpression(tokens, options::HumanReadableSExpressions.HrseReadOptions)
consume(tokens)
expressions = Expression[]
dotexpr = false
dotpos = 0
dotline = 0
while tokentype(peek(tokens)) != RPAREN
if !isnothing(stripindent(tokens))
continue
end
if tokentype(peek(tokens)) == EOF
throw(HrseSyntaxException("Unexpected end of file", tokenline(peek(tokens)), tokenpos(peek(tokens))))
end
if tokentype(peek(tokens)) == DOT
dotexpr = true
dotpos = tokenpos(peek(tokens))
dotline = tokenline(peek(tokens))
consume(tokens)
continue
end
push!(expressions, parseexpression(tokens, options))
end
consume(tokens)
if dotexpr
if length(expressions) != 2
throw(HrseSyntaxException("Expected exactly two expressions surrounding dot in list", dotline, dotpos))
end
return DotExpression(expressions[1], expressions[2])
end
return ListExpression(expressions)
end
function parsestringexpression(tokens, options::HumanReadableSExpressions.HrseReadOptions)
token = consume(tokens)
if token.multiline
lines = StringToken[token]
while tokentype(peek(tokens)) == STRING
nexttoken = consume(tokens)
push!(lines, nexttoken)
if nexttoken.lastline
break
end
end
dropfirstline = isempty(lines[1].value)
if dropfirstline
lines = lines[2:end]
indent = tokens.indentlevel.indent
linevalues = [i.value for i in lines]
if all(startswith.(linevalues, indent))
indentlen = length(indent)
return StringExpression(join([i[indentlen+1:end] for i in linevalues], '\n'))
end
end
return StringExpression(join([i.value for i in lines], '\n'))
end
return StringExpression(token.value)
end
function parseboolexpression(tokens, options::HumanReadableSExpressions.HrseReadOptions)
token = consume(tokens)
return BoolExpression(tokentype(token) == TRUE)
end
function parseimodelineexpression(tokens, options::HumanReadableSExpressions.HrseReadOptions)
expressions = Expression[]
while tokentype(peek(tokens)) == INDENT
consume(tokens)
end
dotexpr = false
dotpos = 0
dotline = 0
while tokentype(peek(tokens)) != INDENT && tokentype(peek(tokens)) != EOF && tokentype(peek(tokens)) != RPAREN
if tokentype(peek(tokens)) == DOT
dotexpr = true
dotpos = tokenpos(peek(tokens))
dotline = tokenline(peek(tokens))
consume(tokens)
continue
end
push!(expressions, parseexpression(tokens, options))
end
if dotexpr
if length(expressions) != 2
throw(HrseSyntaxException("Expected exactly two expressions surrounding dot in list", dotline, dotpos))
end
return DotExpression(expressions[1], expressions[2])
end
if length(expressions) == 1
return expressions[1]
end
return ListExpression(expressions)
end
function translate(expression::ListExpression, options::HumanReadableSExpressions.HrseReadOptions)
[translate(e, options) for e in expression.expressions]
end
function translate(expression::DotExpression, options::HumanReadableSExpressions.HrseReadOptions)
translate(expression.left, options) => translate(expression.right, options)
end
function translate(expression::StringExpression, ::HumanReadableSExpressions.HrseReadOptions)
expression.string
end
function translate(expression::BoolExpression, ::HumanReadableSExpressions.HrseReadOptions)
expression.value
end
function translate(expression::CommentExpression, options::HumanReadableSExpressions.HrseReadOptions)
return HumanReadableSExpressions.CommentedElement(translate(expression.expression, options),expression.comments)
end
function translate(expression::NumberExpression, ::HumanReadableSExpressions.HrseReadOptions)
expression.value
end
end | HumanReadableSExpressions | https://github.com/lukebemish/HumanReadableSExpressions.jl.git |
|
[
"BSD-3-Clause"
]
| 0.1.2 | 7cade784ab71f8a594597382852143e5f25af0e7 | code | 9932 | module Printer
import ..HumanReadableSExpressions
import ..HumanReadableSExpressions: HrsePrintOptions
import ..Literals
import ..Structures
import StructTypes: StructType
import Base.Unicode
isprimitive(obj) = false
isprimitive(::Integer) = true
isprimitive(::AbstractFloat) = true
isprimitive(::Bool) = true
isprimitive(::Symbol) = true
isprimitive(::AbstractString) = true
function pretty(io::IO, obj::AbstractVector, options::HrsePrintOptions, indent::Integer, imode::Bool; kwargs...)
prettyiter(io, obj, options, indent, imode; kwargs...)
end
function prettyiter(io::IO, obj, options::HrsePrintOptions, indent::Integer, imode::Bool; noparenprimitive=false, root=false, kwargs...)
if all(isprimitive(i) for i in obj)
values = [begin
i = IOBuffer()
pretty(i, j, options, indent, imode)
String(take!(i))
end for j in obj]
if length(values) == 0 || sum(length.(values)) + length(values) - 1 <= options.inlineprimitives
if !noparenprimitive || length(obj) <= 1
print(io, '(')
end
print(io, join(values, ' '))
if !noparenprimitive || length(obj) <= 1
print(io, ')')
end
return
else
if !imode
print(io, '(')
end
first = true
for i in values
if !root || !first
println(io)
print(io, options.indent^(indent))
else
first = false
end
print(io, i)
end
end
else
if !imode
print(io, '(')
end
first = true
for i in obj
if !root || !first
println(io)
print(io, options.indent^(indent))
else
first = false
end
pretty(io, i, options, indent+1, false; noparenprimitive=imode)
end
end
if !imode
println(io)
print(io, options.indent^(indent-1), ')')
end
end
function pretty(io::IO, obj::Pair, options::HrsePrintOptions, indent::Integer, imode::Bool; forcegroup=false, kwargs...)
if options.pairmode == HumanReadableSExpressions.DOT_MODE || forcegroup
print(io, '(')
pretty(io, obj.first, options, indent, false)
print(io, " . ")
pretty(io, obj.second, options, indent, false)
print(io, ')')
elseif options.pairmode == HumanReadableSExpressions.EQUALS_MODE
pretty(io, obj.first, options, indent, false; forcegroup=true)
print(io, " = ")
pretty(io, obj.second, options, indent, false)
elseif options.pairmode == HumanReadableSExpressions.COLON_MODE
pretty(io, obj.first, options, indent, false; forcegroup=true)
print(io, ": ")
pretty(io, obj.second, options, indent, true; allownewlinecomments=false)
end
end
pretty(io::IO, obj::AbstractDict, options::HrsePrintOptions, indent::Integer, imode::Bool; kwargs...) = prettyiter(io, obj, options, indent, imode; kwargs...)
pretty(io::IO, obj::Symbol, options::HrsePrintOptions, indent::Integer, imode::Bool; kwargs...) = primitiveprint(io, obj, options)
pretty(io::IO, obj::AbstractString, options::HrsePrintOptions, indent::Integer, imode::Bool; kwargs...) = primitiveprint(io, obj, options)
pretty(io::IO, obj::Integer, options::HrsePrintOptions, indent::Integer, imode::Bool; kwargs...) = primitiveprint(io, obj, options)
pretty(io::IO, obj::AbstractFloat, options::HrsePrintOptions, indent::Integer, imode::Bool; kwargs...) = primitiveprint(io, obj, options)
pretty(io::IO, obj::Bool, options::HrsePrintOptions, indent::Integer, imode::Bool; kwargs...) = primitiveprint(io, obj, options)
function pretty(io::IO, obj::T, options::HrsePrintOptions, indent::Integer, imode::Bool; kwargs...) where T
translated = Structures.serialize(StructType(T), obj, options)
pretty(io, translated, options, indent, imode; kwargs...)
end
function pretty(io::IO, obj::HumanReadableSExpressions.CommentedElement, options::HrsePrintOptions, indent::Integer, imode::Bool; allownewlinecomments=true, kwargs...)
lines = split(join(obj.comments, '\n'), '\n')
if allownewlinecomments && length(lines) == 1 && !isprimitive(obj.element)
println(io, "; ", lines[1])
print(io, options.indent^(indent-1))
else
count = 1
disallowed = Iterators.flatten((length(i) for i in findall(r"(?![^;]);*\)", line)) for line in lines)
while count in disallowed
count += 1
end
first = true
print(io, '(', ';'^count, ' ')
for line in lines
if !first
println(io)
print(io, options.indent^(indent-1), ' '^(count+2))
else
first = false
end
print(io, line)
end
print(io, ' ', ';'^count, ')')
if allownewlinecomments
println(io)
print(io, options.indent^(indent-1))
else print(io, ' ') end
end
pretty(io, obj.element, options, indent, imode)
end
needsspace(obj::AbstractVector, dot::Bool) = false
needsspace(obj::AbstractDict, dot::Bool) = false
needsspace(obj::Pair, dot::Bool) = false
condensed(io::IO, obj::AbstractVector, options::HrsePrintOptions) = denseiter(io, obj, options)
function denseiter(io::IO, obj, options::HrsePrintOptions)
print(io, '(')
first = true
for i in obj
t = translate(i, options)
if !first && needsspace(t, false)
print(io, ' ')
else
first = false
end
condensed(io, t, options)
end
print(io, ')')
end
function condensed(io::IO, obj::Pair, options::HrsePrintOptions)
print(io, '(')
tfirst = translate(obj.first, options)
tsecond = translate(obj.second, options)
condensed(io, tfirst, options)
print(io, needsspaceafter(tfirst) ? ' ' : "", ".", needsspace(tsecond, true) ? ' ' : "")
condensed(io, tsecond, options)
print(io, ')')
end
condensed(io::IO, obj::AbstractDict, options::HrsePrintOptions) = denseiter(io, obj, options)
condensed(io::IO, obj::Symbol, options::HrsePrintOptions) = primitiveprint(io, obj, options)
needsspace(obj::Symbol, dot::Bool) = !dot && Literals.issymbol(string(obj))
condensed(io::IO, obj::AbstractString, options::HrsePrintOptions) = primitiveprint(io, obj, options)
needsspace(obj::AbstractString, dot::Bool) = !dot && Literals.issymbol(string(obj))
condensed(io::IO, obj::Integer, options::HrsePrintOptions) = primitiveprint(io, obj, options)
needsspace(obj::Integer, dot::Bool) = true
condensed(io::IO, obj::AbstractFloat, options::HrsePrintOptions) = primitiveprint(io, obj, options)
needsspace(obj::AbstractFloat, dot::Bool) = true
condensed(io::IO, obj::Bool, options::HrsePrintOptions) = primitiveprint(io, obj, options)
needsspace(obj::Bool, dot::Bool) = !dot
translate(obj::AbstractDict, options::HrsePrintOptions) = obj
translate(obj::Pair, options::HrsePrintOptions) = obj
translate(obj::AbstractVector, options::HrsePrintOptions) = obj
translate(obj::Symbol, options::HrsePrintOptions) = obj
translate(obj::AbstractString, options::HrsePrintOptions) = obj
translate(obj::Integer, options::HrsePrintOptions) = obj
translate(obj::AbstractFloat, options::HrsePrintOptions) = obj
translate(obj::Bool, options::HrsePrintOptions) = obj
translate(obj::HumanReadableSExpressions.CommentedElement, options::HrsePrintOptions) = obj
translate(obj::T, options::HrsePrintOptions) where T = Structures.serialize(StructType(T), obj, options)
function primitiveprint(io::IO, obj::Symbol, options::HrsePrintOptions)
primitiveprint(io, string(obj), options)
end
function primitiveprint(io::IO, obj::AbstractString, options::HrsePrintOptions)
if Literals.issymbol(string(obj))
print(io, obj)
else
print(io, '"')
for c in obj
if haskey(ESCAPES, c)
print(io, '\\', ESCAPES[c])
elseif iscntrl(c) && c != '\t'
print(io, escapesingle(c))
else
print(io, c)
end
end
print(io, '"')
end
end
function primitiveprint(io::IO, obj::Integer, options::HrsePrintOptions)
print(io, string(obj, base=10))
end
function primitiveprint(io::IO, obj::AbstractFloat, options::HrsePrintOptions)
if isinf(obj)
print(io, obj < 0 ? "-#inf" : "#inf")
elseif isnan(obj)
print(io, "#nan")
else
print(io, string(obj))
end
end
function primitiveprint(io::IO, obj::Bool, options::HrsePrintOptions)
print(io, obj ? "#t" : "#f")
end
function condensed(io::IO, obj::HumanReadableSExpressions.CommentedElement, options::HrsePrintOptions)
if options.comments
for comment in obj.comments
count = 1
disallowed = [length(i) for i in findall(r"(?![^;]);*\)", comment)]
while count in disallowed
count += 1
end
print(io, '(', ';'^count, startswith(comment,';') ? ' ' : "", comment, endswith(comment,';') ? ' ' : "", ';'^count, ')')
end
end
condensed(io, translate(obj.element, options), options)
end
condensed(io, obj::T, options::HrsePrintOptions) where T = condensed(io, translate(obj, options), options)
needsspace(obj::HumanReadableSExpressions.CommentedElement, dot::Bool) = false
needsspaceafter(obj) = needsspace(obj, true)
needsspaceafter(obj::HumanReadableSExpressions.CommentedElement) = needsspace(obj.element, true)
const escapesingle(c) = if c <= Char(0o777)
"\\" * string(UInt32(c), base=8, pad=3)
else
"\\u{$(string(UInt32(c), base=16))}"
end
const ESCAPES = Dict(
'\n' => "n",
'\t' => "t",
'\r' => "r",
'\b' => "b",
'\f' => "f",
'\v' => "v",
'\a' => "a",
'\e' => "e",
'"' => "\"",
'\\' => "\\",
)
end | HumanReadableSExpressions | https://github.com/lukebemish/HumanReadableSExpressions.jl.git |
|
[
"BSD-3-Clause"
]
| 0.1.2 | 7cade784ab71f8a594597382852143e5f25af0e7 | code | 6802 | module Structures
import ..HumanReadableSExpressions: HrseReadOptions, HrsePrintOptions
import StructTypes
import StructTypes: StructType, NumberType, BoolType, numbertype, StringType, construct, DictType, ArrayType, Mutable, OrderedStruct, UnorderedStruct, NullType, CustomStruct, AbstractType
function deserialize(::StructType, obj, ::Type{T}, options::HrseReadOptions)::T where T
throw(ArgumentError("Cannot translate object of type $(typeof(obj)) to type $T"))
end
function deserialize(::NumberType, obj::Number, ::Type{T}, options::HrseReadOptions)::T where T
n = convert(numbertype(T), obj)
return construct(T, n)
end
function deserialize(::BoolType, obj::Bool, ::Type{T}, options::HrseReadOptions)::T where T
return construct(T, obj)
end
function deserialize(::StringType, obj::String, ::Type{T}, options::HrseReadOptions)::T where T
return construct(T, obj)
end
function deserialize(::DictType, obj::Vector, ::Type{T}, options::HrseReadOptions)::T where T
dict = Dict(obj)
return construct(T, dict)
end
function deserialize(::DictType, obj::Pair, ::Type{T}, options::HrseReadOptions)::Pair where T <: Pair
return Pair(
pairfirst(T, obj, options),
pairsecond(T, obj, options)
)
end
function pairfirst(::Type{<:Pair}, obj::Pair, options::HrseReadOptions)
return obj.first
end
function pairfirst(::Type{<:Pair{A}}, obj::Pair, options::HrseReadOptions) where A
return deserialize(StructType(A), obj.first, A, options)
end
function pairfirst(::Type{<:Pair{A,B}}, obj::Pair, options::HrseReadOptions) where {A,B}
return deserialize(StructType(A), obj.first, A, options)
end
function pairsecond(::Type{<:Pair}, obj::Pair, options::HrseReadOptions)
return obj.second
end
function pairsecond(::Type{<:Pair{A,B}}, obj::Pair, options::HrseReadOptions) where {A,B}
return deserialize(StructType(B), obj.second, B, options)
end
function deserialize(::ArrayType, obj::Vector, ::Type{T}, options::HrseReadOptions)::T where T
if Base.IteratorEltype(T) == Base.HasEltype()
eltype = Base.eltype(T)
return construct(T, map(x -> deserialize(StructType(eltype), x, eltype, options), obj))
end
return construct(T, obj)
end
function deserialize(::NullType, obj::Vector, ::Type{T}, options::HrseReadOptions)::T where T
if length(obj) > 0
throw(ArgumentError("Null must be represented by an empty list!"))
end
return T()
end
function deserialize(::Mutable, obj::Vector, ::Type{T}, options::HrseReadOptions)::T where T
dict = try
Dict(obj)
catch
throw(ArgumentError("Vector contains non-dict entries!"))
end
out = T()
for (k, v) in dict
fn = deserialize(StructType(Symbol), k, Symbol, options)
applied = StructTypes.applyfield!(out, fn) do i, name, ft
deserialize(StructType(ft), v, ft, options)
end
if !applied
throw(ArgumentError("Cannot apply field $fn to type $T"))
end
end
return out
end
function deserialize(::OrderedStruct, obj::Vector, ::Type{T}, options::HrseReadOptions)::T where T
dict = try
Dict(obj)
catch
throw(ArgumentError("Vector contains non-dict entries!"))
end
out = []
StructTypes.foreachfield(T) do i, name, ft
if haskey(dict, String(name))
push!(out, deserialize(StructType(ft), dict[String(name)], ft, options))
end
end
return construct(T, out...)
end
function deserialize(::UnorderedStruct, obj::Vector, ::Type{T}, options::HrseReadOptions)::T where T
dict = try
Dict(obj)
catch
throw(ArgumentError("Vector contains non-dict entries!"))
end
out = []
StructTypes.foreachfield(T) do i, name, ft
if haskey(dict, String(name))
push!(out, deserialize(StructType(ft), dict[String(name)], ft, options))
else
push!(out, nothing)
end
end
return construct(T, out...)
end
function deserialize(::CustomStruct, obj::Vector, ::Type{T}, options::HrseReadOptions)::T where T
lowered = StructTypes.lowertype(T)
return construct(T, deserialize(StructType(lowered), obj, lowered, options))
end
function deserialize(::AbstractType, obj::Vector, ::Type{T}, options::HrseReadOptions)::T where T
key = String(StructTypes.subtypekey(T))
i = findfirst(j -> (j isa Pair && first(j) == key), obj)
v = obj[i]
translated = deserialize(StructType(Symbol), last(v), Symbol, options)
if !haskey(StructTypes.subtypes(T), translated)
throw(ArgumentError("Cannot translate object to to type $T with key $translated"))
end
newtype = StructTypes.subtypes(T)[translated]
return deserialize(StructType(newtype), obj, newtype, options)
end
function serialize(::StructType, obj, options::HrsePrintOptions)
throw(ArgumentError("Cannot translate object of type $(typeof(obj)) to a serializable form"))
end
function serialize(::NumberType, obj::T, options::HrsePrintOptions) where T
return numbertype(T)(obj)
end
function serialize(::BoolType, obj, options::HrsePrintOptions)
return Bool(obj)
end
function serialize(::StringType, obj, options::HrsePrintOptions)
return string(obj)
end
function serialize(::DictType, obj, options::HrsePrintOptions)
return [serialize(StructType(typeof(k)), k, options) => serialize(StructType(typeof(v)), v, options) for (k, v) in StructTypes.keyvaluepairs(obj)]
end
function serialize(::DictType, obj::Pair, options::HrsePrintOptions)
return serialize(StructType(typeof(obj.first)), obj.first, options) => serialize(StructType(typeof(obj.second)), obj.second, options)
end
function serialize(::ArrayType, obj, options::HrsePrintOptions)
return [serialize(StructType(typeof(v)), v, options) for v in obj]
end
function serialize(::NullType, obj, options::HrsePrintOptions)
return []
end
function serialize(::Mutable, obj, options::HrsePrintOptions)
out = []
StructTypes.foreachfield(obj) do i, name, ft, v
push!(out, serialize(StructType(Symbol), name, options) => serialize(StructType(ft), v, options))
end
return out
end
function serialize(::OrderedStruct, obj, options::HrsePrintOptions)
out = []
StructTypes.foreachfield(obj) do i, name, ft, v
push!(out, serialize(StructType(ft), v, options))
end
return out
end
function serialize(::UnorderedStruct, obj, options::HrsePrintOptions)
out = []
StructTypes.foreachfield(obj) do i, name, ft, v
push!(out, serialize(StructType(Symbol), name, options) => serialize(StructType(ft), v, options))
end
return out
end
function serialize(::CustomStruct, obj, options::HrsePrintOptions)
lowered = StructTypes.lower(obj)
return serialize(StructType(lowered), lowered, options)
end
end | HumanReadableSExpressions | https://github.com/lukebemish/HumanReadableSExpressions.jl.git |
|
[
"BSD-3-Clause"
]
| 0.1.2 | 7cade784ab71f8a594597382852143e5f25af0e7 | code | 7605 | using HumanReadableSExpressions
import HumanReadableSExpressions: Parser.HrseSyntaxException
import StructTypes
using Test
struct Foo
a::Int
b::String
end
mutable struct Bar
a::Pair{Foo, String}
b::Vector{Foo}
c::Float64
Bar() = new()
end
abstract type Vehicle end
struct Car <: Vehicle
type::String
make::String
model::String
seatingCapacity::Int
topSpeed::Float64
end
struct Truck <: Vehicle
type::String
make::String
model::String
payloadCapacity::Float64
end
StructTypes.StructType(::Type{Vehicle}) = StructTypes.AbstractType()
StructTypes.StructType(::Type{Car}) = StructTypes.Struct()
StructTypes.StructType(::Type{Truck}) = StructTypes.Struct()
StructTypes.subtypekey(::Type{Vehicle}) = :type
StructTypes.subtypes(::Type{Vehicle}) = (car=Car, truck=Truck)
StructTypes.StructType(::Type{Foo}) = StructTypes.UnorderedStruct()
StructTypes.StructType(::Type{Bar}) = StructTypes.Mutable()
hrse = """
(; this is great for testing edge cases ;;)
and comment formats;)
(;;; mostly just various multiline comments...
;) ;;;;) ;;;)
a: 1
b: 2
c:
(;;; should still parse ;;;)
1
2
(; ;)
3
(; ;)
d: 4
e:
(
1
2
3
4
5:
6
7
8
)
(
a:
1
2)
b
c
"""
# This will hit an absurd number of edge cases all at once, it turns out...
@test readhrse(hrse) == Pair{String}["a" => 1, "b" => 2, "c" => [1, 2, 3], "d" => 4, "e" => [[1, 2, 3, 4, 5 => [6, 7, 8]], ["a" => [1, 2]], "b", "c"]]
hrse = """
a=1
b=2
c=3
(
a=1
(b . 2)
c: 3
)
"""
@test readhrse(hrse) == ["a" => 1, "b" => 2, "c" => 3, ["a" => 1, "b" => 2, "c" => 3]]
car = """
(
type: car
make: Mercedes-Benz
model: S500
seatingCapacity: 5
topSpeed: 250.1
)
"""
@test readhrse(car, type=Vehicle, options=HrseReadOptions(extensions=[HumanReadableSExpressions.DENSE])) == Car("car", "Mercedes-Benz", "S500", 5, 250.1)
@test ashrse(Truck("truck", "Ford", "F-150", 1000.0), HrsePrintOptions()) == """
type: truck
make: Ford
model: F-150
payloadCapacity: 1000.0
"""
@test ashrse(Truck("truck", "Ford", "F-150", 1000.0), HrsePrintOptions(pairmode = HumanReadableSExpressions.DOT_MODE)) == """
(type . truck)
(make . Ford)
(model . F-150)
(payloadCapacity . 1000.0)
"""
@test ashrse(Truck("truck", "Ford", "F-150", 1000.0), HrsePrintOptions(pairmode = HumanReadableSExpressions.EQUALS_MODE)) == """
type = truck
make = Ford
model = F-150
payloadCapacity = 1000.0
"""
foo = Foo(1, "foo")
bar = Bar()
bar.a = Foo(2, "bar") => "bar"
bar.b = [Foo(3, "baz"), Foo(4, "qux")]
bar.c = 3.14
@test ashrse(foo, HrsePrintOptions(extensions=[HumanReadableSExpressions.DENSE])) == """
(
a: 1
b: foo
)
"""
@test ashrse(bar, HrsePrintOptions(pairmode=HumanReadableSExpressions.CONDENSED_MODE)) == "(a.(((a. 2)(b.bar)).bar))(b.(((a. 3)(b.baz))((a. 4)(b.qux))))(c. 3.14)"
@test readhrse("((a.(((a. 2)(b.bar)).bar))(b.(((a. 3)(b.baz))((a. 4)(b.qux))))(c. 3.14))", type=Bar, options=HrseReadOptions(extensions=[HumanReadableSExpressions.DENSE])) !== nothing
hrse = """
; Yeah, let's just test every type imaginable...
1
999999999999999999999999
1e1
1e+1
1e-1
.1e1
1.
1.e1
1.1
1.1e1
1_.1
1._1
1_000
#t
#f
#inf
-#inf
+#inf
#nan
"test"
test
"""
@test all((parsed = readhrse(hrse)) .== [1, big"999999999999999999999999", 10.0, 10.0, 0.1, 1.0, 1.0, 10.0, 1.1, 11.0, 1.1, 1.1, 1000, true, false, Inf, -Inf, Inf, NaN, "test", "test"] .|| (parsed .=== NaN))
@test ashrse([1,1.0,1e20,Inf,-Inf,NaN,"test"," test",true,false], HrsePrintOptions()) == """
1
1.0
1.0e20
#inf
-#inf
#nan
test
" test"
#t
#f
"""
# Other int encodings
hrse = """
0xAbC10
0b11010
-10
-0x10
+20
"""
@test readhrse(hrse) == [282574486936592, 26, -10, -16, 20]
hrse = """
"\\u{1234}\\012\\03abc\\a\\e\\\\\\"\\r\\n\\f\\b\\v\\t\\u{12}"
"""
@test readhrse(hrse, options=HrseReadOptions(extensions=[HumanReadableSExpressions.DENSE])) == "\u1234\n\x03abc\a\e\\\"\r\n\f\b\v\t\x12"
# Encoding
dict = Dict([
:a => 1,
:b => 2,
:c => [:a=>2,:b=>2]])
@test ashrse(dict, HrsePrintOptions(pairmode=HumanReadableSExpressions.DOT_MODE)) == """
(a . 1)
(b . 2)
(c . (
(a . 2)
(b . 2)
))
"""
@test ashrse(dict, HrsePrintOptions(pairmode=HumanReadableSExpressions.EQUALS_MODE)) == """
a = 1
b = 2
c = (
a = 2
b = 2
)
"""
@test ashrse(dict, HrsePrintOptions(pairmode=HumanReadableSExpressions.CONDENSED_MODE)) == "(a. 1)(b. 2)(c.((a. 2)(b. 2)))"
@test ashrse(dict, HrsePrintOptions(pairmode=HumanReadableSExpressions.COLON_MODE)) == """
a: 1
b: 2
c:\40
a: 2
b: 2
"""
@test ashrse(dict, HrsePrintOptions(trailingnewline=false)) == """
a: 1
b: 2
c:\40
a: 2
b: 2"""
@test ashrse(dict, HrsePrintOptions(indent=" ")) == """
a: 1
b: 2
c:\40
a: 2
b: 2
"""
@test ashrse(dict, HrsePrintOptions(indent="\t")) == """
a: 1
b: 2
c:\40
\ta: 2
\tb: 2
"""
@test ashrse(dict, HrsePrintOptions(extensions=[HumanReadableSExpressions.DENSE])) == """
(
a: 1
b: 2
c:\40
a: 2
b: 2
)
"""
longlist = [:a => repeat([1], 20)]
shortlist = [:a => repeat([1], 5)]
@test ashrse(longlist, HrsePrintOptions()) == """
a:\40
1
1
1
1
1
1
1
1
1
1
1
1
1
1
1
1
1
1
1
1
"""
@test ashrse(shortlist, HrsePrintOptions()) == """
a: (1 1 1 1 1)
"""
@test ashrse(shortlist, HrsePrintOptions(inlineprimitives=3)) == """
a:\40
1
1
1
1
1
"""
@test ashrse(longlist, HrsePrintOptions(inlineprimitives=40)) == """
a: (1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1)
"""
@test readhrse("""
a: \"\"\"
abc
\"\"\"
""") == ["a" => "abc\n"]
@test readhrse("""
a: \"\"\"
abc\"\"\"
""") == ["a" => "abc"]
@test readhrse("""
a: \"\"\" abc
def\"\"\"
""") == ["a" => " abc\n def"]
@test readhrse("""
a: \"\"\"
""-\"\"\"""") == ["a" => "\"\"-"]
@test readhrse("""
a: \"\"\"
a \\
b \\
c\"\"\"
""") == ["a" => "a b c"]
@test readhrse("""
a:
\tb: \"\"\"
\tabc\"\"\"
""") == ["a" => ["b" => "abc"]]
# Test string literals
@test readhrse("a-b") == ["a-b"]
@test_throws HrseSyntaxException readhrse("-ax")
@test_throws HrseSyntaxException readhrse(".a")
@test_throws HrseSyntaxException readhrse("0stuff")
@test readhrse("_1 not-a-number") == [["_1", "not-a-number"]]
@test_throws "HrseSyntaxException: Invalid literal '#invalid-literal' at line 1, column 1" readhrse("#invalid-literal")
@test_throws HrseSyntaxException readhrse("\"\a\"")
@test_throws HrseSyntaxException readhrse("a\u00AD")
@test_throws HrseSyntaxException readhrse("a\U2019")
@test readhrse("a^") == ["a^"]
@test readhrse("a1") == ["a1"]
@test_throws "HrseSyntaxException: Unterminated string at line 1, column 4" readhrse("""
a: \" stuff
""")
@test_throws "HrseSyntaxException: Unterminated string at line 1, column 4" readhrse("""
a: \"\"\" stuff
""")
@test_throws "HrseSyntaxException: Unterminated multiline comment at line 1, column 1" readhrse("""
(;
""")
@test_throws "HrseSyntaxException: Unterminated multiline comment at line 1, column 1" readhrse("""
(; ;;)
""")
@test_throws "HrseSyntaxException: Unterminated multiline comment at line 1, column 1" readhrse("""
(;; ;)
""")
@test_throws "HrseSyntaxException: Invalid number '200000' at line 1, column 1" readhrse("200000", options=HrseReadOptions(integertypes=[Int8]))
@test_throws HrseSyntaxException readhrse("\"abc\"def")
@test_throws HrseSyntaxException readhrse("\"abc\"\"def\"")
# Test indents
@test_throws "HrseSyntaxException: Unexpected indent level at line 4, column 1" readhrse("""
a:
a
b
c
d
""")
@test readhrse("""
a:
(
1 2 3
)
(
4 5 6
)
(;
7 8 9
;)
""") == ["a"=>[[1,2,3],[4,5,6]]] | HumanReadableSExpressions | https://github.com/lukebemish/HumanReadableSExpressions.jl.git |
|
[
"BSD-3-Clause"
]
| 0.1.2 | 7cade784ab71f8a594597382852143e5f25af0e7 | docs | 1004 | # HumanReadableSExpressions.jl
[](https://lukebemish.dev/HumanReadableSExpressions.jl/dev)
[](https://lukebemish.dev/HumanReadableSExpressions.jl/stable)
[](https://github.com/lukebemish/HumanReadableSExpressions.jl/actions/workflows/CI.yml?query=branch%3Amain)
[](https://codecov.io/gh/lukebemish/HumanReadableSExpressions.jl)
### Human Readable S-Expressions
HumanReadableSExpressions.jl is a Julia package for reading and writing files written in [HRSE](https://lukebemish.dev/hrse), or Human Readable
S-Expressions, which are a human-readable format for representing data and configuration files that is equivalent and
and interchangeable with s-expressions.
| HumanReadableSExpressions | https://github.com/lukebemish/HumanReadableSExpressions.jl.git |
|
[
"BSD-3-Clause"
]
| 0.1.2 | 7cade784ab71f8a594597382852143e5f25af0e7 | docs | 1003 | # HumanReadableSExpressions.jl Documentation
```@contents
Depth = 4
```
## Getting Started
HumanReadableSExpressions.jl is a Julia package for reading and writing files written in [HRSE](https://lukebemish.dev/hrse),
or Human Readable S-Expressions, which are a human-readable format for representing data and configuration files that is
equivalent and and interchangeable with s-expressions.
HumanReadableSExpressions.jl provides two main functions, `readhrse` and `writehrse`, which read and write HRSE files,
respectively. Both functions support custom serialization and deserialization of types using StructTypes.jl.
## API
```@docs
readhrse
HrseReadOptions
writehrse
ashrse
HrsePrintOptions
HumanReadableSExpressions.CommentedElement
HumanReadableSExpressions.Extension
HumanReadableSExpressions.DENSE
HumanReadableSExpressions.PairMode
HumanReadableSExpressions.CONDENSED_MODE
HumanReadableSExpressions.DOT_MODE
HumanReadableSExpressions.EQUALS_MODE
HumanReadableSExpressions.COLON_MODE
```
| HumanReadableSExpressions | https://github.com/lukebemish/HumanReadableSExpressions.jl.git |
|
[
"MIT"
]
| 0.2.13 | 0f7960bdb9439853e5912e905b008d703c179838 | code | 5609 | module MLSuiteBase
using JSON, SHA, Glob
import ScikitLearnBase: is_classifier
export @grid, gridparams, reset!, istree, isrnn, is_ranker, support_multiclass, signone, modelhash, available_memory, myparam, gendir
export @gc, @staticvar, @staticdef, @redirect, logerr, @tryonce, prefix, suffix
reset!(m) = nothing
istree(m) = false
isrnn(m) = false
support_multiclass(m) = is_classifier(m)
is_ranker(m) = false
modelhash(m) = hash(m)
gridparams(grid, combine = Iterators.product) =
unique(vec([merge(v...) for v in combine(gridparams.(reverse(grid))...)]))
function gridparams((k, vs)::Pair{String})
if haskey(ENV, k)
v = try eval(Meta.parse(ENV[k])) catch e ENV[k] end
[Dict{String, Any}(k => v)]
else
[Dict{String, Any}(k => v) for v in vs]
end
end
gridparams(grid::Vector{<:Vector}) = mapreduce(gridparams, vcat, grid)
Base.sign(x::Real, Θ) = ifelse(x < -Θ, oftype(x, -1), ifelse(x > Θ, one(x), zero(x)))
function signone(x::Real, Θ = zero(x))
y = sign(x, Θ)
!iszero(y) && return y
rand([-one(x), one(x)])
end
function embed_include(file, mod)
if isfile(file)
lines = readlines(file)
else
lines = split(file, '\n')
end
for (n, line) in enumerate(lines)
startswith(line, '#') && continue
m = match(r"(?<=include\().*?(?=\))", line)
isnothing(m) && continue
src = mod.eval(Meta.parse(m.match))
src = isabspath(src) ? src : joinpath(dirname(file), src)
lines[n] = embed_include(src, mod)
end
join(lines, '\n')
end
macro grid(n, ex)
params = __module__.eval(ex)
file = string(__source__.file)
if !isfile(file) || isinteractive() ||
get(ENV, "GRID_ENABLE", "1") == "0"
return params[1]
end
line = __source__.line
lines = split(embed_include(file, __module__), '\n')
root = "job_" * splitext(basename(file))[1]
project = "#!/bin/bash\nexport JULIA_PROJECT=$(Base.load_path()[1])\n"
for param in rand(params, n)
dir = mkpath(joinpath(root, bytes2hex(sha1(json(param)))))
open(joinpath(dir, "param.json"), "w") do io
JSON.print(io, param, 4)
end
write(joinpath(dir, "julia.sh"), project, raw"""
source ~/.bashrc
cd $(dirname $0)
[ -f done ] && exit 0
julia main.jl 2>&1 | tee julia.out
[ $? == 0 ] && touch done
""")
write(joinpath(dir, "mpi.sh"), project, raw"""
cd $(dirname $0)
[ -f done ] && exit 0
mpirun julia main.jl 2>&1 | tee mpi.out
[ $? == 0 ] && touch done
""")
write(joinpath(dir, "srun.sh"), project, raw"""
[ -f done ] && exit 0
srun julia main.jl
[ $? == 0 ] && touch done
""")
var_name = match(r"(.+?)(?=\s+\=)", lines[line]).match
lines[line] = "$var_name = $param"
write(joinpath(dir, "main.jl"), join(lines, '\n'))
foreach(sh -> chmod(sh, 0o775), glob("*.sh", dir))
end
return :(exit())
end
function myparam(params)
if haskey(ENV, "SLURM_ARRAY_TASK_ID")
i = parse(Int, "0" * ENV["SLURM_ARRAY_TASK_ID"]) + 1
elseif haskey(ENV, "PBS_ARRAYID")
i = parse(Int, "0" * ENV["PBS_ARRAYID"]) + 1
else
i = 1
end
i <= length(params) ? params[i] : exit(0)
end
function gendir(param)
dir = mkpath(bytes2hex(sha1(json(param))))
open(joinpath(dir, "param.json"), "w") do io
JSON.print(io, param, 4)
end
return dir
end
function available_memory()
if Sys.iswindows()
Sys.free_memory() / 1024^3
else
regex = r"MemAvailable:\s+(\d+)\s+kB"
meminfo = read("/proc/meminfo", String)
mem = match(regex, meminfo).captures[1]
parse(Int, mem) / 1024^2
end
end
function JSON.lower(a)
if nfields(a) > 0
JSON.Writer.CompositeTypeWrapper(a)
else
string(a)
end
end
macro gc(exs...)
Expr(:block, [:($ex = 0) for ex in exs]..., :(@eval GC.gc())) |> esc
end
macro staticvar(init)
var = gensym()
__module__.eval(:(const $var = $init))
var = esc(var)
quote
global $var
$var
end
end
macro staticdef(ex)
name, T = ex.args[1].args
ref = Ref{__module__.eval(T)}()
set = Ref(false)
:($(esc(name)) = if $set[]
$ref[]
else
$ref[] = $(esc(ex))
$set[] = true
$ref[]
end)
end
macro redirect(src, ex)
src = src == :devnull ? "/dev/null" : src
quote
io = open($(esc(src)), "a")
o, e = stdout, stderr
redirect_stdout(io)
redirect_stderr(io)
local res = nothing
try
res = $(esc(ex))
sleep(0.01)
finally
flush(io)
close(io)
redirect_stdout(o)
redirect_stderr(e)
end
res
end
end
function logerr(e, comment = "", fname = "try.err", mode = "a")
e isa InterruptException && rethrow()
io = IOBuffer()
print(io, '-'^15, gethostname(), '-'^15, '\n', e)
bt = stacktrace(catch_backtrace())
showerror(io, e, bt)
println(io)
str = join(Iterators.take(String(take!(io)), 10000))
str = join(map(l -> join(Iterators.take(l, 400)), split(str, '\n')), '\n')
println(stdout, comment)
println(stdout, str)
flush(stdout)
open(fname, mode) do fid
println(fid, comment)
println(fid, str)
end
end
macro tryonce(ex)
ex = Expr(:try, ex, :e, :(logerr(e)))
return esc(ex)
end
prefix(str) = String(split(str, '.')[1])
suffix(str) = String(split(str, '.')[end])
end | MLSuiteBase | https://github.com/AStupidBear/MLSuiteBase.jl.git |
|
[
"MIT"
]
| 0.2.13 | 0f7960bdb9439853e5912e905b008d703c179838 | code | 28 | using MLSuiteBase
using Test | MLSuiteBase | https://github.com/AStupidBear/MLSuiteBase.jl.git |
|
[
"MIT"
]
| 0.2.13 | 0f7960bdb9439853e5912e905b008d703c179838 | docs | 302 | # MLSuiteBase
[](https://github.com/AStupidBear/MLSuiteBase.jl/actions)
[](https://codecov.io/gh/AStupidBear/MLSuiteBase.jl)
| MLSuiteBase | https://github.com/AStupidBear/MLSuiteBase.jl.git |
|
[
"MIT"
]
| 0.3.8 | d6f73792bf7f162a095503f47427c66235c0c772 | code | 693 | using POMDPs
using ARDESPOT
using POMDPToolbox
using POMDPModels
using ProgressMeter
T = 50
N = 100
pomdp = BabyPOMDP()
bounds = IndependentBounds(reward(pomdp, true, false)/(1-discount(pomdp)), 0.0)
solver = DESPOTSolver(epsilon_0=0.1,
bounds=bounds,
T_max=Inf,
rng=MersenneTwister(4)
)
rsum = 0.0
fwc_rsum = 0.0
@showprogress for i in 1:N
planner = solve(solver, pomdp)
sim = RolloutSimulator(max_steps=T, rng=MersenneTwister(i))
fwc_sim = deepcopy(sim)
rsum += simulate(sim, pomdp, planner)
fwc_rsum += simulate(sim, pomdp, FeedWhenCrying())
end
@show rsum/N
@show fwc_rsum/N
| ARDESPOT | https://github.com/JuliaPOMDP/ARDESPOT.jl.git |
|
[
"MIT"
]
| 0.3.8 | d6f73792bf7f162a095503f47427c66235c0c772 | code | 502 | using Gallium
using POMDPs
using ARDESPOT
using ProfileView
using POMDPModels
pomdp = BabyPOMDP()
bounds = IndependentBounds(reward(pomdp, true, false)/(1-discount(pomdp)), 0.0)
solver = DESPOTSolver(epsilon_0=0.0,
bounds=bounds,
rng=MersenneTwister(4),
random_source=MersenneSource(500, 1, MersenneTwister(4))
)
p = solve(solver, pomdp)
b0 = initial_state_distribution(pomdp)
@enter ARDESPOT.build_despot(p, b0)
| ARDESPOT | https://github.com/JuliaPOMDP/ARDESPOT.jl.git |
|
[
"MIT"
]
| 0.3.8 | d6f73792bf7f162a095503f47427c66235c0c772 | code | 484 | using POMDPs
using ARDESPOT
using ProfileView
using POMDPModels
pomdp = BabyPOMDP()
bounds = IndependentBounds(reward(pomdp, true, false)/(1-discount(pomdp)), 0.0)
solver = DESPOTSolver(epsilon_0=0.0,
bounds=bounds,
rng=MersenneTwister(4),
random_source=MersenneSource(500, 1, MersenneTwister(4))
)
p = solve(solver, pomdp)
b0 = initial_state_distribution(pomdp)
D = ARDESPOT.build_despot(p, b0)
| ARDESPOT | https://github.com/JuliaPOMDP/ARDESPOT.jl.git |
|
[
"MIT"
]
| 0.3.8 | d6f73792bf7f162a095503f47427c66235c0c772 | code | 1135 | using Revise
using POMDPs
using ARDESPOT
using ProfileView
using POMDPModels
using BenchmarkTools
using POMDPToolbox
pomdp = BabyPOMDP()
# bounds = IndependentBounds(DefaultPolicyLB(FeedWhenCrying()), 0.0)
bounds = IndependentBounds(DefaultPolicyLB(RandomPolicy(pomdp, rng=MersenneTwister(14))), 0.0)
# bounds = IndependentBounds(reward(pomdp, false, true)/(1-discount(pomdp)), 0.0)
solver = DESPOTSolver(epsilon_0=0.0,
K=500,
D=50,
bounds=bounds,
T_max=Inf,
max_trials=100,
rng=MersenneTwister(4),
# random_source=FastMersenneSource(500, 10)
random_source=MemorizingSource(500, 90, MersenneTwister(5))
)
p = solve(solver, pomdp)
b0 = initial_state_distribution(pomdp)
println("starting first")
@time ARDESPOT.build_despot(p, b0)
@time ARDESPOT.build_despot(p, b0)
@time ARDESPOT.build_despot(p, b0)
@time ARDESPOT.build_despot(p, b0)
Profile.clear()
D = @profile for i in 1:20
ARDESPOT.build_despot(p, b0)
end
ProfileView.view()
| ARDESPOT | https://github.com/JuliaPOMDP/ARDESPOT.jl.git |
|
[
"MIT"
]
| 0.3.8 | d6f73792bf7f162a095503f47427c66235c0c772 | code | 518 | using Revise
using TraceCalls
using POMDPs
using ARDESPOT
using ProfileView
using POMDPModels
pomdp = BabyPOMDP()
bounds = IndependentBounds(reward(pomdp, true, false)/(1-discount(pomdp)), 0.0)
solver = DESPOTSolver(epsilon_0=0.0,
bounds=bounds,
rng=MersenneTwister(4),
random_source=MersenneSource(500, 1, MersenneTwister(4))
)
p = solve(solver, pomdp)
b0 = initial_state_distribution(pomdp)
@trace ARDESPOT.build_despot(p, b0)
| ARDESPOT | https://github.com/JuliaPOMDP/ARDESPOT.jl.git |
|
[
"MIT"
]
| 0.3.8 | d6f73792bf7f162a095503f47427c66235c0c772 | code | 666 | using Revise
using POMDPs
using ARDESPOT
using ProfileView
using POMDPModels
pomdp = BabyPOMDP()
bounds = IndependentBounds(reward(pomdp, true, false)/(1-discount(pomdp)), 0.0)
solver = DESPOTSolver(epsilon_0=0.0,
K=100,
lambda=0.01,
bounds=bounds,
max_trials=3,
rng=MersenneTwister(4),
random_source=FastMersenneSource(1000, 1)
)
@show solver.lambda
p = solve(solver, pomdp)
b0 = initial_state_distribution(pomdp)
@show b0
@time D = ARDESPOT.build_despot(p, b0)
@show action(p, b0)
println(TreeView(D, 1, 90))
| ARDESPOT | https://github.com/JuliaPOMDP/ARDESPOT.jl.git |
|
[
"MIT"
]
| 0.3.8 | d6f73792bf7f162a095503f47427c66235c0c772 | code | 3958 | #LightDark from POMDPModels.jl, modified for discrete binned observations
using Distributions
# A one-dimensional light-dark problem, originally used to test MCVI
# A very simple POMDP with continuous state and observation spaces.
# maintained by @zsunberg
"""
LightDark1D_DO
A one-dimensional light dark problem. The goal is to be near 0. Observations are noisy measurements of the position.
Model
-----
-3-2-1 0 1 2 3
...| | | | | | | | ...
G S
Here G is the goal. S is the starting location
"""
mutable struct LightDark1D_DO{F<:Function} <: POMDPs.POMDP{LightDark1DState,Int,Float64}
discount_factor::Float64
correct_r::Float64
incorrect_r::Float64
step_size::Float64
movement_cost::Float64
sigma::F
grid::Tuple{Float64, Float64}
grid_step::Float64
grid_len::Int
end
default_sigma(x::Float64) = abs(x - 5)/sqrt(2) + 1e-2
function LightDark1D_DO(;grid = collect(-10:20/40:10))
return LightDark1D_DO(0.9, 10.0, -10.0, 1.0, 0.0, default_sigma,(first(grid),last(grid)),round(grid[2]-grid[1],digits=4),length(grid))
end
POMDPs.discount(p::LightDark1D_DO) = p.discount_factor
POMDPs.isterminal(::LightDark1D_DO, act::Int64) = act == 0
POMDPs.isterminal(::LightDark1D_DO, s::LightDark1DState) = s.status < 0
POMDPs.actions(::LightDark1D_DO) = -1:1
POMDPs.initialstate(pomdp::LightDark1D_DO) = POMDPModels.LDNormalStateDist(2, 3)
POMDPs.initialobs(m::LightDark1D_DO, s) = observation(m, s)
struct DiscreteNormal
dist::Normal{Float64}
g_first::Float64
g_end::Float64
step::Float64
end
function DiscreteNormal(p::LightDark1D_DO,dist::Normal{Float64})
return DiscreteNormal(dist,p.grid[1],p.grid[2],p.grid_step)
end
function Base.rand(rng::AbstractRNG,dist::DiscreteNormal)
val = rand(rng,dist.dist)
# @show val
# @show ceil((val-dist.g_first)/dist.step)*dist.step+dist.g_first
return ceil((val-dist.g_first)/dist.step)*dist.step+dist.g_first
end
function Distributions.pdf(dist::DiscreteNormal,x::Float64)
@assert ceil(round((x-dist.g_first)/dist.step),digits=8)%1.0 == 0.0
discx = x
val = 0.0
if x <= dist.g_first
val = cdf(dist.dist,discx)
elseif x >= dist.g_end
val = 1.0-cdf(dist.dist,discx-dist.step)
else
val = cdf(dist.dist,discx)-cdf(dist.dist,discx-dist.step)
end
return val
end
function POMDPs.observation(p::LightDark1D_DO, sp::LightDark1DState)
return DiscreteNormal(p,Normal(sp.y, p.sigma(sp.y)))
end
# function POMDPs.observation(p::LightDark1D_DO, sp::LightDark1DState)
# dist = Normal(sp.y, p.sigma(sp.y))
# o_vals = zeros(p.grid_len)
# old_cdf = 0.0
# grid = collect(p.grid[1]:p.grid_step:p.grid[2])
# for (i,g) in enumerate(grid)
# if i == 1
# old_cdf = cdf(dist,g)
# o_vals[i] = old_cdf
# elseif i == p.grid_len
# o_vals[end] = 1.0-old_cdf
# else
# new_cdf = cdf(dist,g)
# o_vals[i] = new_cdf-old_cdf
# old_cdf = new_cdf
# end
# end
# # @assert all(o_vals .>= 0)
# # @assert abs(sum(o_vals)-1.0) < 0.0001
# return SparseCat(grid,o_vals)
# end
function POMDPs.transition(p::LightDark1D_DO, s::LightDark1DState, a::Int)
if a == 0
return Deterministic(LightDark1DState(-1, s.y+a*p.step_size))
else
return Deterministic(LightDark1DState(s.status, s.y+a*p.step_size))
end
end
function POMDPs.reward(p::LightDark1D_DO, s::LightDark1DState, a::Int)
if s.status < 0
return 0.0
elseif a == 0
if abs(s.y) < 1
return p.correct_r
else
return p.incorrect_r
end
else
return -p.movement_cost*a
end
end
convert_s(::Type{A}, s::LightDark1DState, p::LightDark1D_DO) where A<:AbstractArray = eltype(A)[s.status, s.y]
convert_s(::Type{LightDark1DState}, s::A, p::LightDark1D_DO) where A<:AbstractArray = LightDark1DState(Int64(s[1]), s[2]) | ARDESPOT | https://github.com/JuliaPOMDP/ARDESPOT.jl.git |
|
[
"MIT"
]
| 0.3.8 | d6f73792bf7f162a095503f47427c66235c0c772 | code | 2016 | #Benchmarks for ARDESPOT.jl PR No. 32 (Use OrderedDict)
#Note: Uses HistoryRecorder instead of calls to action directly. This may add additional noise.
using Pkg
Pkg.activate(".")
using ARDESPOT
Pkg.activate("pr32_benchmarks")
using BenchmarkTools
using POMDPs
using POMDPModels
using Random
using POMDPTools
using ParticleFilters
##Baby Tests
pomdp = BabyPOMDP()
pomdp.discount = 1.0
bds = IndependentBounds(DefaultPolicyLB(FeedWhenCrying()), 0.0)
solver = DESPOTSolver(bounds=bds,T_max=Inf,max_trials=1000,rng=MersenneTwister(5))
planner = solve(solver, pomdp)
hr = HistoryRecorder(max_steps=2)
println("BabyPOMDP ===================================")
display(@benchmark simulate(hr, pomdp, planner))
println("")
##Tiger Tests
pomdp = TigerPOMDP()
solver = DESPOTSolver(bounds=(-20.0, 0.0),T_max=Inf,max_trials=1000,rng=MersenneTwister(5))
planner = solve(solver, pomdp)
hr = HistoryRecorder(max_steps=3)
println("Tiger ===================================")
display(@benchmark simulate(hr, pomdp, planner))
println("")
##LightDark POMDP
include("LD_disc_o.jl")
pomdp = LightDark1D_DO(;grid = collect(-10:20/40:10))
lb_pol = FunctionPolicy(b->-1)
bds = IndependentBounds(DefaultPolicyLB(lb_pol), pomdp.correct_r,check_terminal=true)
solver = DESPOTSolver(bounds=bds,T_max=Inf,max_trials=1000,rng=MersenneTwister(5))
planner = solve(solver, pomdp)
hr = HistoryRecorder(max_steps=2)
println("LightDark D.O. - 40 Obs ===================================")
display(@benchmark simulate(hr, pomdp, planner))
println("")
##LD 2
pomdp = LightDark1D_DO(;grid = collect(-10:20/100:10))
lb_pol = FunctionPolicy(b->-1)
bds = IndependentBounds(DefaultPolicyLB(lb_pol), pomdp.correct_r,check_terminal=true)
solver = DESPOTSolver(bounds=bds,T_max=Inf,max_trials=1000,rng=MersenneTwister(5))
planner = solve(solver, pomdp)
hr = HistoryRecorder(max_steps=2)
println("LightDark D.O. - 100 Obs ===================================")
display(@benchmark simulate(hr, pomdp, planner))
println("")
#Add RockSample???
"done" | ARDESPOT | https://github.com/JuliaPOMDP/ARDESPOT.jl.git |
|
[
"MIT"
]
| 0.3.8 | d6f73792bf7f162a095503f47427c66235c0c772 | code | 1591 | #LD Policies for Bounds
struct LDPolicy{M<:POMDP,S}<:Policy
m::M
particle_dict::Dict{S,Float64}
act::Int64
planner::DPWPlanner
end
function LDPolicy(m::LightDark1D{S}) where S
solver = DPWSolver(n_iterations=50, depth=20, exploration_constant=5.0)
planner = solve(solver, UnderlyingMDP(m))
return LDPolicy(m,Dict{S,Float64}(),-1,planner)
end
##Tyler's map_bel:
@inline function get_incr!(h::Dict{K,V}, key::K, v) where {K,V} # modified from dict.jl
index = Base.ht_keyindex2!(h, key)
v = convert(V, v)
if index > 0
h.age += 1
return h.vals[index] += 1
end
age0 = h.age
if h.age != age0
index = Base.ht_keyindex2!(h, key)
end
if index > 0
h.age += 1
@inbounds h.keys[index] = key
@inbounds h.vals[index] = v
else
@inbounds Base._setindex!(h, v, key, -index)
end
return v
end
function map_bel(b::AbstractParticleBelief, pol)
empty!(pol.particle_dict)
dict = pol.particle_dict
max_o = 0.0
# max_state = first(particles(b))
max_state = pol.m.state1
for (p,w) in weighted_particles(b)
n = get_incr!(dict, p, w)
if n > max_o
max_o = n
max_state = p
end
end
return max_state
end
function POMDPs.action(policy::LDPolicy,s::Union{ParticleCollection,ScenarioBelief})
max_p = map_bel(s,policy)
return POMDPs.action(policy,max_p)
end
function POMDPs.action(policy::LDPolicy,s::POMDPModels.LDNormalStateDist)
max_p = map_bel(s,policy)
return POMDPs.action(policy,max_p)
end | ARDESPOT | https://github.com/JuliaPOMDP/ARDESPOT.jl.git |
|
[
"MIT"
]
| 0.3.8 | d6f73792bf7f162a095503f47427c66235c0c772 | code | 3774 | module ARDESPOT
using POMDPs
using Parameters
using CPUTime
using ParticleFilters
using D3Trees
using Random
using Printf
using POMDPTools
using BasicPOMCP # for ExceptionRethrow and NoDecision
import BasicPOMCP.default_action
import Random.rand
export
DESPOTSolver,
DESPOTPlanner,
DESPOTRandomSource,
MemorizingSource,
MemorizingRNG,
ScenarioBelief,
previous_obs,
default_action,
NoGap,
IndependentBounds,
FullyObservableValueUB,
DefaultPolicyLB,
bounds,
init_bounds,
lbound,
ubound,
init_bound,
ReportWhenUsed
# include("random.jl")
include("random_2.jl")
"""
DESPOTSolver(<keyword arguments>)
Implementation of the ARDESPOT solver trying to closely match the pseudo code of:
http://bigbird.comp.nus.edu.sg/m2ap/wordpress/wp-content/uploads/2017/08/jair14.pdf
Each field may be set via keyword argument. The fields that correspond to algorithm
parameters match the definitions in the paper exactly.
# Fields
- `epsilon_0`
- `xi`
- `K`
- `D`
- `lambda`
- `T_max`
- `max_trials`
- `bounds`
- `default_action`
- `rng`
- `random_source`
- `bounds_warnings`
- `tree_in_info`
Further information can be found in the field docstrings (e.g.
`?DESPOTSolver.xi`)
"""
@with_kw mutable struct DESPOTSolver <: Solver
"The target gap between the upper and the lower bound at the root of the partial DESPOT."
epsilon_0::Float64 = 0.0
"The rate of target gap reduction."
xi::Float64 = 0.95
"The number of sampled scenarios."
K::Int = 500
"The maximum depth of the DESPOT."
D::Int = 90
"Reguluarization constant."
lambda::Float64 = 0.01
"The maximum online planning time per step."
T_max::Float64 = 1.0
"The maximum number of trials of the planner."
max_trials::Int = typemax(Int)
"A representation for the upper and lower bound on the discounted value (e.g. `IndependentBounds`)."
bounds::Any = IndependentBounds(-1e6, 1e6)
"""A default action to use if algorithm fails to provide an action because of an error.
This can either be an action object, i.e. `default_action=1` if `actiontype(pomdp)==Int` or a function `f(pomdp, b, ex)` where b is the belief and ex is the exception that caused the planner to fail.
"""
default_action::Any = ExceptionRethrow()
"A random number generator for the internal sampling processes."
rng::MersenneTwister = MersenneTwister(rand(UInt32))
"A source for random numbers in scenario rollout"
random_source::DESPOTRandomSource = MemorizingSource(K, D, rng)
"If true, sanity checks on the provided bounds are performed."
bounds_warnings::Bool = true
"If true, a reprenstation of the constructed DESPOT is returned by POMDPTools.action_info."
tree_in_info::Bool = false
end
include("scenario_belief.jl")
include("default_policy_sim.jl")
include("bounds.jl")
struct DESPOTPlanner{P<:POMDP, B, RS<:DESPOTRandomSource, RNG<:AbstractRNG} <: Policy
sol::DESPOTSolver
pomdp::P
bounds::B
rs::RS
rng::RNG
end
function DESPOTPlanner(sol::DESPOTSolver, pomdp::POMDP)
bounds = init_bounds(sol.bounds, pomdp, sol)
rng = deepcopy(sol.rng)
rs = deepcopy(sol.random_source)
Random.seed!(rs, rand(rng, UInt32))
return DESPOTPlanner(deepcopy(sol), pomdp, bounds, rs, rng)
end
include("tree.jl")
include("planner.jl")
include("pomdps_glue.jl")
include("visualization.jl")
include("exceptions.jl")
end # module
| ARDESPOT | https://github.com/JuliaPOMDP/ARDESPOT.jl.git |
|
[
"MIT"
]
| 0.3.8 | d6f73792bf7f162a095503f47427c66235c0c772 | code | 5184 | init_bounds(bounds, pomdp, sol) = bounds
init_bounds(t::Tuple, pomdp, sol) = (init_bound(first(t), pomdp, sol), init_bound(last(t), pomdp, sol))
bounds(f::Function, pomdp::POMDP, b::ScenarioBelief) = f(pomdp, b)
bounds(t::Tuple, pomdp::POMDP, b::ScenarioBelief) = (lbound(t[1], pomdp, b), ubound(t[2], pomdp, b))
function bounds_sanity_check(pomdp::POMDP, sb::ScenarioBelief, L_0, U_0)
if L_0 > U_0
@warn("L_0 ($L_0) > U_0 ($U_0) |ϕ| = $(length(sb.scenarios))")
@info("Try e.g. `IndependentBounds(l, u, consistency_fix_thresh=1e-5)`.", maxlog=1)
end
if all(isterminal(pomdp, s) for s in particles(sb))
if L_0 != 0.0 || U_0 != 0.0
error(@sprintf("If all states are terminal, lower and upper bounds should be zero (L_0=%-10.2g, U_0=%-10.2g). (try IndependentBounds(l, u, check_terminal=true))", L_0, U_0))
end
end
if isinf(L_0) || isnan(L_0)
@warn("L_0 = $L_0. Infinite bounds are not supported.")
end
if isinf(U_0) || isnan(U_0)
@warn("U_0 = $U_0. Infinite bounds are not supported.")
end
end
"""
IndependentBounds(lower, upper, check_terminal=false, consistency_fix_thresh=0.0)
Specify lower and upper bounds that are independent of each other (the most common case).
# Keyword Arguments
- `check_terminal::Bool=false`: if true, then if all the states in the belief are terminal, the upper and lower bounds will be overridden and set to 0.
- `consistency_fix_thresh::Float64=0.0`: if `upper < lower` and `upper >= lower-consistency_fix_thresh`, then `upper` will be bumped up to `lower`.
"""
struct IndependentBounds{L, U}
lower::L
upper::U
check_terminal::Bool
consistency_fix_thresh::Float64
end
function IndependentBounds(l, u;
check_terminal=false,
consistency_fix_thresh=0.0)
return IndependentBounds(l, u, check_terminal, consistency_fix_thresh)
end
function bounds(bounds::IndependentBounds, pomdp::POMDP, b::ScenarioBelief)
if bounds.check_terminal && all(isterminal(pomdp, s) for s in particles(b))
return (0.0, 0.0)
end
l = lbound(bounds.lower, pomdp, b)
u = ubound(bounds.upper, pomdp, b)
if u < l && u >= l-bounds.consistency_fix_thresh
u = l
end
return (l,u)
end
function init_bounds(bounds::IndependentBounds, pomdp::POMDP, sol::DESPOTSolver)
return IndependentBounds(init_bound(bounds.lower, pomdp, sol),
init_bound(bounds.upper, pomdp, sol),
bounds.check_terminal,
bounds.consistency_fix_thresh
)
end
init_bound(bound, pomdp, sol) = bound
ubound(n::Number, pomdp, b) = convert(Float64, n)
lbound(n::Number, pomdp, b) = convert(Float64, n)
ubound(f::Function, pomdp, b) = f(pomdp, b)
lbound(f::Function, pomdp, b) = f(pomdp, b)
# Value if Fully Observed Under a Policy
struct FullyObservableValueUB{P<:Union{Solver, Policy}}
p::P
end
ubound(ub::FullyObservableValueUB, pomdp::POMDP, b::ScenarioBelief) = mean(value(ub.p, s) for s in particles(b)) # assumes that all are weighted equally
function init_bound(ub::FullyObservableValueUB{S}, pomdp::POMDP, sol::DESPOTSolver) where S <: Solver
return FullyObservableValueUB(solve(ub.p, pomdp))
end
# Default Policy Lower Bound
"""
DefaultPolicyLB(policy; max_depth=nothing, final_value=(m,x)->0.0)
DefaultPolicyLB(solver; max_depth=nothing, final_value=(m,x)->0.0)
A lower bound calculated by running a default policy on the scenarios in a belief.
# Keyword Arguments
- `max_depth::Union{Nothing,Int}=nothing`: max depth to run the simulation. The depth of the belief will be automatically subtracted so simulations for the bound will be run for `max_depth-b.depth` steps. If `nothing`, the solver's max depth will be used.
- `final_value=(m,x)->0.0`: a function (or callable object) that specifies an additional value to be added at the end of the simulation when `max_depth` is reached. This function will be called with two arguments, a `POMDP`, and a `ScenarioBelief`. It will not be called when the states in the belief are terminal.
"""
struct DefaultPolicyLB{P<:Union{Solver, Policy}, D<:Union{Nothing,Int}, T}
policy::P
max_depth::D
final_value::T
end
function DefaultPolicyLB(policy_or_solver::T;
max_depth=nothing,
final_value=(m,x)->0.0) where T <: Union{Solver, Policy}
return DefaultPolicyLB(policy_or_solver, max_depth, final_value)
end
function lbound(lb::DefaultPolicyLB, pomdp::POMDP, b::ScenarioBelief)
rsum = branching_sim(pomdp, lb.policy, b, lb.max_depth-b.depth, lb.final_value)
return rsum/length(b.scenarios)
end
function init_bound(lb::DefaultPolicyLB{S}, pomdp::POMDP, sol::DESPOTSolver) where S <: Solver
policy = solve(lb.policy, pomdp)
return init_bound(DefaultPolicyLB(policy, lb.max_depth, lb.final_value), pomdp, sol)
end
function init_bound(lb::DefaultPolicyLB{P}, pomdp::POMDP, sol::DESPOTSolver) where P <: Policy
max_depth = something(lb.max_depth, sol.D)
return DefaultPolicyLB(lb.policy, max_depth, lb.final_value)
end
| ARDESPOT | https://github.com/JuliaPOMDP/ARDESPOT.jl.git |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.