id
int64 393k
2.82B
| repo
stringclasses 68
values | title
stringlengths 1
936
| body
stringlengths 0
256k
⌀ | labels
stringlengths 2
508
| priority
stringclasses 3
values | severity
stringclasses 3
values |
---|---|---|---|---|---|---|
352,925,924 | angular | downgrade component doesn't forward errors to $exceptionHandler | <!--
PLEASE HELP US PROCESS GITHUB ISSUES FASTER BY PROVIDING THE FOLLOWING INFORMATION.
ISSUES MISSING IMPORTANT INFORMATION MAY BE CLOSED WITHOUT INVESTIGATION.
-->
## I'm submitting a...
<!-- Check one of the following options with "x" -->
<pre><code>
[ ] Regression (a behavior that used to work and stopped working in a new release)
[x] Bug report <!-- Please search GitHub for a similar issue or PR before submitting -->
[ ] Performance issue
[ ] Feature request
[ ] Documentation issue or request
[ ] Support request => Please do not submit support request here, instead see https://github.com/angular/angular/blob/master/CONTRIBUTING.md#question
[ ] Other... Please describe:
</code></pre>
## Current behavior
<!-- Describe how the issue manifests. -->
Seems like errors that occur in component constructor are resulting in unhandled promise rejection error:
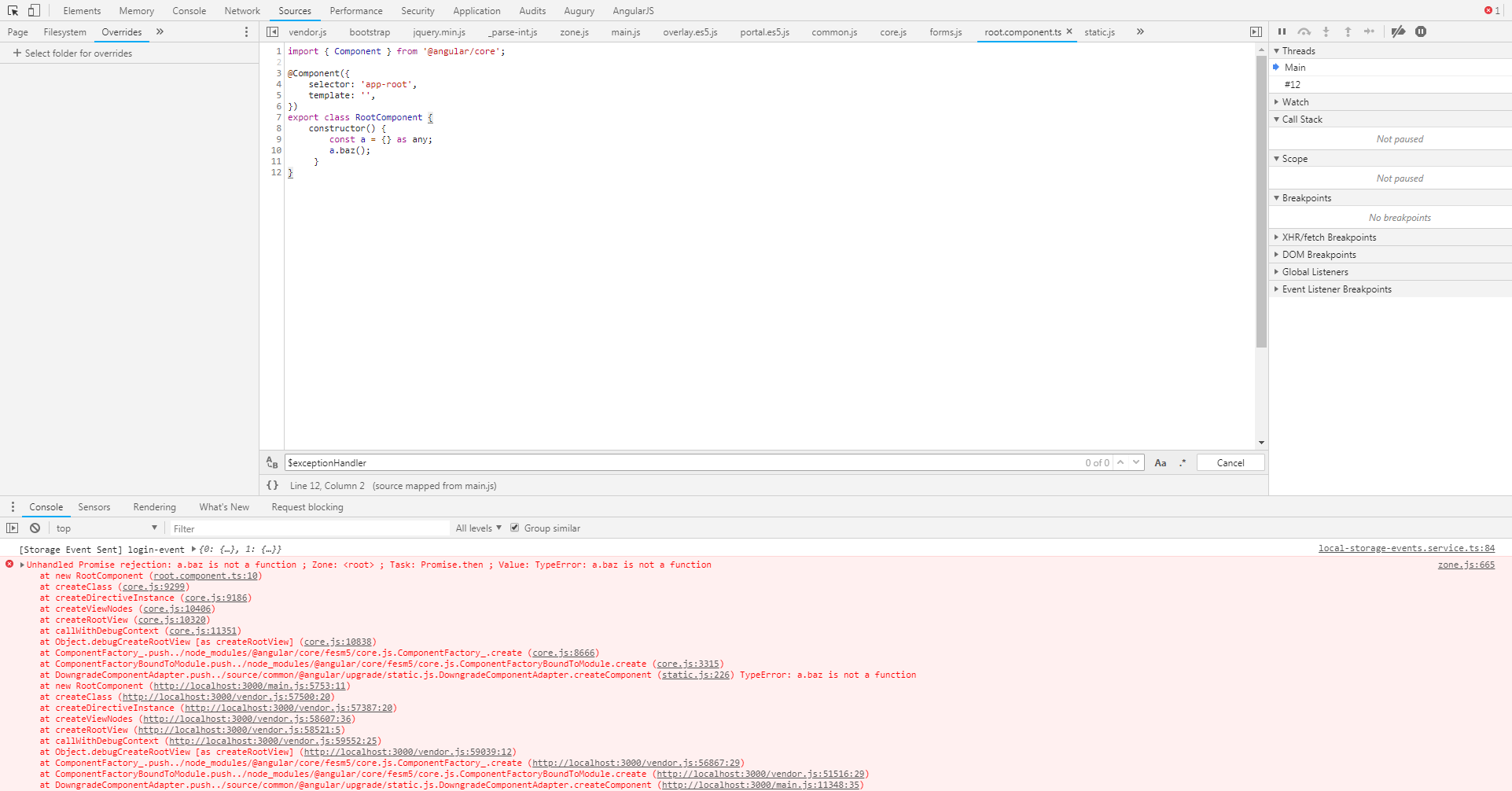
## Expected behavior
<!-- Describe what the desired behavior would be. -->
Instead of being logged by zone.js (which is nice!) it should be forwarded to angular.js $exceptionHandler and handled according to the project setup - in this case custom a logger service
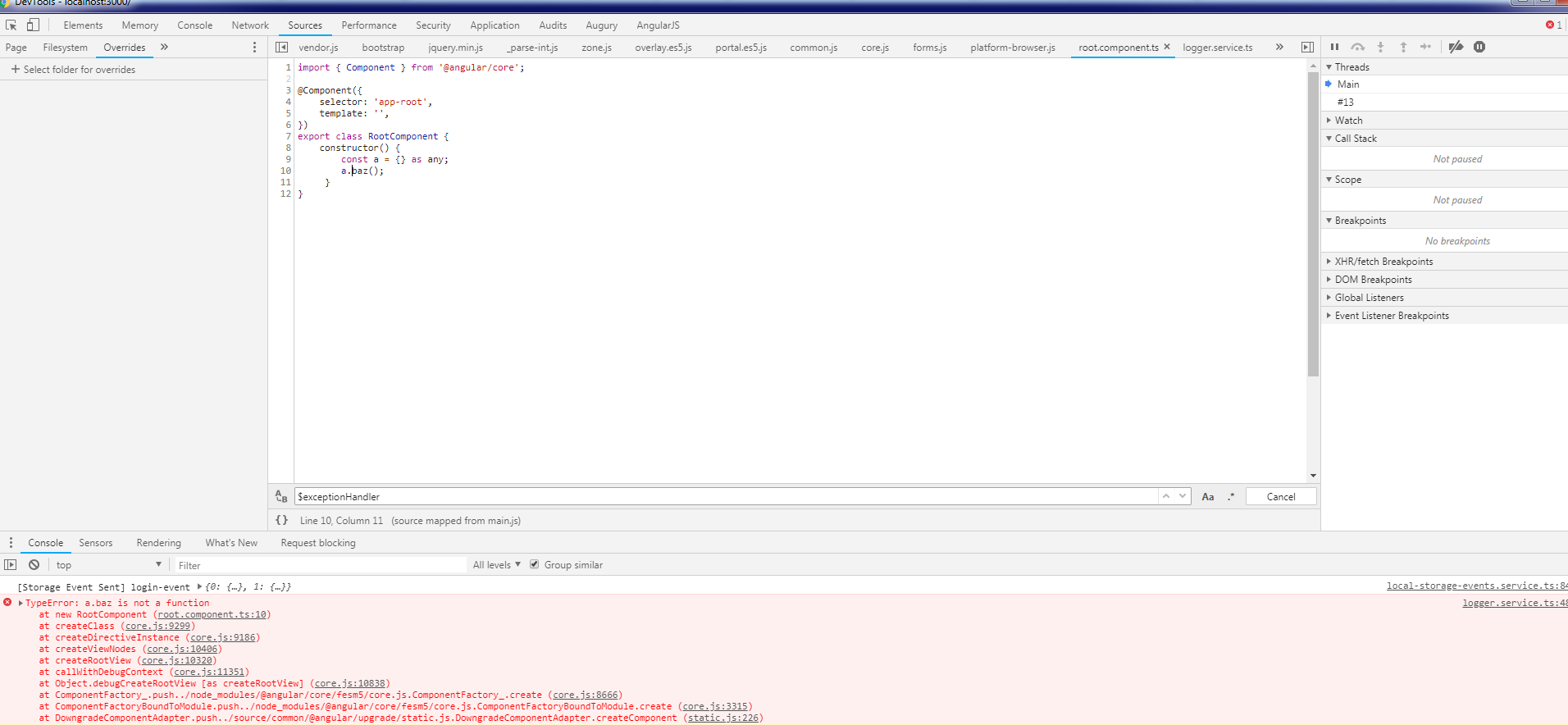
## What is the motivation / use case for changing the behavior?
<!-- Describe the motivation or the concrete use case. -->
This way errors would be forwarded to some implementation of $log or custom $exception handler and could be tracked / reported.
The fix is simple: include `$exceptionHandler` to [dependencies](https://github.com/angular/angular/blob/master/packages/upgrade/src/common/downgrade_component.ts#L157) and add `.catch($exceptionHandler)` in [here](https://github.com/angular/angular/blob/master/packages/upgrade/src/common/downgrade_component.ts#L146)
## other
We are still using `upgrade/static`
EDIT: For suggested change to work correctly, `ParentInjectorPromise` should also return an object with a noop `catch` method | type: bug/fix,area: upgrade,state: confirmed,P4 | low | Critical |
352,955,106 | go | cmd/compile: optimise ranges over string(byteSlice) | Take these two benchmarks: https://play.golang.org/p/QI4BxUq8MGp
The first code is cleaner, more idiomatic, and easier to write/maintain. The second is much trickier, and I'm not even sure I wrote it correctly.
Lucky for us, the first tends to perform about the same or slightly better in terms of time:
```
$ go test -bench=. -benchmem
goos: linux
goarch: amd64
pkg: mvdan.cc/p
BenchmarkIdiomatic-4 500000 2389 ns/op 2688 B/op 1 allocs/op
BenchmarkManual-4 500000 2645 ns/op 0 B/op 0 allocs/op
PASS
ok mvdan.cc/p 2.578s
```
However, as one can see, it still incurs an extra allocation. I'm not sure why that is - via `go test -gcflags=-m`, I can see `./f_test.go:16:27: BenchmarkIdiomatic string(Input) does not escape`.
We have optimized other common string conversion patterns, such as `switch string(byteSlice)`
in 566e3e074c089568412a44a8d315c2881cfd8e8f, and I believe `someMap[string(byteSlice)]` was optimized too.
Would it be possible to get rid of this allocation somehow? My knowledge of the runtime and compiler is a bit limited, but I'd think that it is possible.
As for a real use case - there's a few occurrences in the standard library that could be greatly simplified. For example, in `encoding/json` we'd remove ten lines of tricky code:
```
diff --git a/src/encoding/json/decode.go b/src/encoding/json/decode.go
index 2e734fb39e..fa09b85aab 100644
--- a/src/encoding/json/decode.go
+++ b/src/encoding/json/decode.go
@@ -1210,20 +1210,11 @@ func unquoteBytes(s []byte) (t []byte, ok bool) {
// then no unquoting is needed, so return a slice of the
// original bytes.
r := 0
- for r < len(s) {
- c := s[r]
- if c == '\\' || c == '"' || c < ' ' {
- break
- }
- if c < utf8.RuneSelf {
- r++
- continue
- }
- rr, size := utf8.DecodeRune(s[r:])
- if rr == utf8.RuneError && size == 1 {
+ for i, c := range string(s) {
+ if c == '\\' || c == '"' || c < ' ' || c == utf8.RuneError {
break
}
- r += size
+ r = i
}
if r == len(s) {
return s, true
```
However, that currently means a bad regression in speed and allocations:
```
name old time/op new time/op delta
CodeDecoder-4 27.3ms ± 1% 31.2ms ± 1% +14.16% (p=0.002 n=6+6)
name old speed new speed delta
CodeDecoder-4 71.1MB/s ± 1% 62.3MB/s ± 1% -12.41% (p=0.002 n=6+6)
name old alloc/op new alloc/op delta
CodeDecoder-4 2.74MB ± 0% 5.13MB ± 0% +87.28% (p=0.002 n=6+6)
name old allocs/op new allocs/op delta
CodeDecoder-4 77.5k ± 0% 184.3k ± 0% +137.72% (p=0.002 n=6+6)
```
I haven't investigated why my microbenchmark is faster when simpler, while json gets so much slower when made simpler.
Any input appreciated. cc @josharian @martisch @randall77 @TocarIP | Performance,NeedsInvestigation,compiler/runtime | low | Critical |
352,970,429 | opencv | reprojectImageTo3D not thread safe |
- OpenCV => 3.4.1:
- Operating System / Platform => Ubuntu 16.04:
- Compiler => GCC 5.5
##### Detailed description
cv::reprojectImageTo3D from calib3d is not thread safe. When used in a multithreaded application, it gives large memory leaks. My current real-time application leaks approx 50 MB/sec, which can be manually traced to this function.
(valgrind does not work due to an AVX512 assertion, even though OpenCV was build while specifically disabling all AVX instructions using -D CPU_BASELINE_DISABLE= AVX512F AVX2 AVX SSE4.1 SSSE3 SSE3 AVX512_SKX. So please dont ask for valgrind output).
Can be easily reproduced in a tbb::pipeline or using tbb::parallel_invoke as shown below.
In my own application the output is ok, only the memory leaks.
##### Steps to reproduce
Run the below code and check the memory usage, which will grow for each call.
// C++ code example
```
#include <opencv2/core.hpp>
#include <opencv2/calib3d.hpp>
#include <tbb/parallel_invoke.h>
#include <iostream>
void testReprojection1()
{
cv::Mat fakeDisparity(1920, 1440, CV_32F);
cv::randn(fakeDisparity, 200, 100);
cv::Mat Q = (cv::Mat_<float>(4, 4) << 1, 0, 0, -960, 0 , 1, 0, -720, 0 , 0, 0, 1500, 0 , 0, 1/150, 0);
cv::Mat output;
cv::reprojectImageTo3D(fakeDisparity, output, Q, false);
}
void testReprojection2()
{
cv::Mat fakeDisparity(1920, 1440, CV_32F);
cv::randn(fakeDisparity, 200, 100);
cv::Mat Q = (cv::Mat_<float>(4, 4) << 1, 0, 0, -960, 0 , 1, 0, -720, 0 , 0, 0, 1500, 0 , 0, 1/150, 0);
cv::Mat output;
cv::reprojectImageTo3D(fakeDisparity, output, Q, false);
}
int main(int fArgc, char **fArgv)
{
try
{
for (int i = 0; i < 1000; ++i)
{
tbb::parallel_invoke(testReprojection1, testReprojection2);
}
}
catch (const std::exception &fEx)
{
std::cerr << "Error: " << fEx.what() << std::endl;
return EXIT_FAILURE;
}
std::cout << "Exiting program" << std::endl;
}
```
| incomplete | low | Critical |
353,081,878 | TypeScript | Missing completion for abstract indexed types | **TypeScript Version:** 5.1.3
**Search Terms:**
missing, suggestion, code completions, language-server, abstract, generic
**Code**
```ts
export abstract class StateMachine {
abstract state: any;
getProperty<K extends keyof this["state"]>(key: K): this["state"][K] {
return this.state[key];
}
}
export class SomeStateMachine extends StateMachine {
state = {
menu: {
login() {
console.log("Menu.login");
},
},
game: {
update() {
console.log("Game.update");
},
},
};
constructor() {
super();
// ⌄--- No code-completions here...!
this.getProperty("menu").login();
}
}
```
**Expected behavior:**
I expected to get suggestions for the `this.getProperty`'s key parameter (used in the constructor).
The suggestion should be "menu" or "game".
**Actual behavior:**
The compiler is able to identify the "correct" keys ("menu" | "game") but not able to suggest them via code completions.
**Playground Link:**
[Playground link](https://www.typescriptlang.org/play?pretty=false&ts=5.1.3#code/IYIwzgLgTsDGEAJYBthjAgyhYECmAsnABYCWAdnggN4CwAUAgqJDPApLngFzPkCeAbgYMmAczwQAClAD2ABzxQI-ADwBpBHgAe+cgBMMAazz9ZAMwQQyYANoAiTvnsBdAHwAKE-17qAlLzWpHaOOM4utuouNKJMCFCSAK5Q5FY2AHROeLbeLsKMCAC+DMX0DChoGJiyALZ42FxEsGSUWrp4BlVhhCQUVHQFWQgAvDEFTHXkibwDcXHIsmIUHn5jc3OwsuRgssh46QtiHvYEHYkHixT2fvnrRQA0sUyFj+MIYsB1M09xifL6XBWazuSC2Oz2FyO9gA4p99n8Ac4bj9nq85i9YoV8rFNttoIl4LIoEDZnEwH8lCtbnEgmB0hJpHJFMp+MdJolrpDlsiCqVSkA)
**Related Issues:**
Found none. | Bug | low | Minor |
353,083,144 | flutter | InkWell and InkResponse not close enough to the real thing | When I briefly tap stuff on native Android, I usually can see the highlight and splash very easily. However, in Flutter, both `InkRipple.splashFactory` and `InkSplash.splashFactory` are slower to show/hide. `InkRipple.splashFactory` is better in my opinion, should be the default, but it's not quite right also.
More importantly, in Android native the splash is shown when I REMOVE my finger, while in Flutter it's shown when I hold my finger down.
I wish splash/highlight were tweaked to obtain the exact same effect of native Android. | framework,f: material design,a: fidelity,f: gestures,customer: crowd,P2,team-design,triaged-design | low | Major |
353,112,733 | opencv | build farm is not testing for CUDA functionalities | The development cycle of CUDA-related functionalities is inefficient and incomplete!
Kindly consider introducing a CUDA-enabled hardware, and making it part of the required tests. | priority: low,category: gpu/cuda (contrib),category: infrastructure,RFC | low | Minor |
353,121,913 | rust | UnixStream does not have connect_timeout as TcpStream does. | https://doc.rust-lang.org/std/net/struct.TcpStream.html#method.connect_timeout
vs
https://doc.rust-lang.org/std/os/unix/net/struct.UnixStream.html
Is this something that could be added directly without an RFC? | T-libs-api,C-feature-request | low | Minor |
353,147,454 | go | x/mobile: unexpected truncation of int type iOS->Go | Please answer these questions before submitting your issue. Thanks!
### What version of Go are you using (`go version`)?
> go version go1.10.3 darwin/amd64
`golang.org/x/mobile` commit `bceb7ef27cc623473a5b664d2a3450576dddff0f`
### Does this issue reproduce with the latest release?
Yep
### What operating system and processor architecture are you using (`go env`)?
```
GOARCH="amd64"
GOBIN=""
GOCACHE="/Users/verm/Library/Caches/go-build"
GOEXE=""
GOHOSTARCH="amd64"
GOHOSTOS="darwin"
GOOS="darwin"
GOPATH="/Users/verm/remix/turntable/amp"
GORACE=""
GOROOT="/usr/local/Cellar/go/1.10.3/libexec"
GOTMPDIR=""
GOTOOLDIR="/usr/local/Cellar/go/1.10.3/libexec/pkg/tool/darwin_amd64"
GCCGO="gccgo"
CC="clang"
CXX="clang++"
CGO_ENABLED="1"
CGO_CFLAGS="-g -O2"
CGO_CPPFLAGS=""
CGO_CXXFLAGS="-g -O2"
CGO_FFLAGS="-g -O2"
CGO_LDFLAGS="-g -O2"
PKG_CONFIG="pkg-config"
GOGCCFLAGS="-fPIC -m64 -pthread -fno-caret-diagnostics -Qunused-arguments -fmessage-length=0 -fdebug-prefix-map=/var/folders/36/92jbhtpj41s6q3w4fcq2v01w0000gn/T/go-build566537997=/tmp/go-build -gno-record-gcc-switches -fno-common"
```
### What did you do?
I had a function that needed to take a timeout, which internally is a `time.Duration` (`int64`). That's not a type I can use in the mobile bindings, so I used an `int` parameter. This gets translated as a `long` in Objective-C. My Obj-C code took an `int64_t` value and passed it as the long parameter of the wrapped Go function.
I then passed an Obj-C `int64_t` value that doesn't fit in a 32-bit int to this function.
### What did you expect to see?
I would expect that Go would see the original 64-bit value unaffected.
### What did you see instead?
Surprisingly, is that even though `long` (in Obj-C) and `int` (in Go) are 64-bit types on these platform (Mac OS X 10.13.6 / iOS simulator and iPhone 7 running iOS 11.4), the result in Go was an `int` holding the lower 32 bits of the original value. Specifically, for an input of `int64_t x = 86400000000000`, the value of the `int` passed to the Go function was `-1857093632`.
In this particular case, the correct choice in retrospect was clearly to use `int64` as the parameter type in Go. This results in a wrapper type in Objective-C of `int64_t`, and everything works fine.
But I am still surprised that `int` would behave in this way on a 64-bit system.
| mobile | low | Critical |
353,152,605 | pytorch | [distributed] Synchronization on CUDA side with MPI backend | ## Issue description
`torch.distributed` with OpenMPI 3.0.0 as backend: when running "blocking" communication commands (e.g. `send`, `recv`, etc. are blocking in MPI), CUDA side is not synchronized. OpenMPI does not do that automatically, PyTorch does not do that automatically. This incurred several timing issues in some of my applications. I think this should be either
- documented: so that let users add things like `torch.cuda.synchronize` properly, or
- synchronized: synchronize the device (`cudaDeviceSynchronize`) before/after MPI blocking communication call.
## System Info
- PyTorch or Caffe2: PyTorch
- How you installed PyTorch (conda, pip, source): source
- Build command you used (if compiling from source): `python setup.py install`
- OS: ubuntu 16.04
- PyTorch version: 0.4.0
- Python version: 3.6.4
- CUDA/cuDNN version: 8.0
- GPU models and configuration: GTX 1080
- GCC version (if compiling from source): 5.4.0
- CMake version: 3.11.1
- Versions of any other relevant libraries: OpenMPI 3.0.0
cc @jlin27 @mruberry @pietern @mrshenli @pritamdamania87 @zhaojuanmao @satgera @rohan-varma @gqchen @aazzolini @xush6528 @osalpekar @jiayisuse @agolynski | oncall: distributed,module: docs,triaged,module: c10d,distributed-backlog | low | Minor |
353,159,916 | TypeScript | Intersection type of abstract classes does not throw error if same member exists in multiple abstract classes | **TypeScript Version:** 3.0.1
**Search Terms:** "Intersection", "abstract"
**Code**
```ts
export type Constructor<T = {}> = new (...args: any[]) => T;
abstract class Outer1 {
abstract same: number;
abstract notSame: string;
}
abstract class Outer2 {
abstract same: number;
}
function Mixin1<TBase extends Constructor>(Base: TBase) {
abstract class Inner1 extends Base { // Same as Outer1
abstract same: number;
}
return Inner1;
}
function Mixin2<TBase extends Constructor>(Base: TBase) {
abstract class Inner2 extends Base { // Same as Outer2
abstract same: number;
}
return Inner2;
}
function rollUp<T>(mixins): new (...args) => T {
return mixins.reduce((acc, mixin) => mixin(acc), class Seed {});
}
class Example extends rollUp<Outer1 & Outer2>([Mixin1, Mixin2]) {
// Compiler will throw error when "notSame" is unimplemented
// Compiler DOES NOT throw error about non-implemented "same" member
}
```
**Expected behavior:**
Compiler should say that `same` is not implemented in regular class
**Actual behavior:**
Compiler does not throw error saying that `same` is not present in example class, despite throwing an error about `notSame`. It will throw an error, however, if the types of `same` are incompatible.
**Playground Link:** https://www.typescriptlang.org/play/#src=export%20type%20Constructor%3CT%20%3D%20%7B%7D%3E%20%3D%20new%20(...args%3A%20any%5B%5D)%20%3D%3E%20T%3B%0D%0A%0D%0Aabstract%20class%20Outer1%20%7B%0D%0A%20%20abstract%20same%3A%20number%3B%0D%0A%20%20abstract%20notSame%3A%20string%3B%0D%0A%7D%0D%0A%0D%0Aabstract%20class%20Outer2%20%7B%0D%0A%20%20abstract%20same%3A%20number%3B%0D%0A%7D%0D%0A%0D%0Afunction%20Mixin1%3CTBase%20extends%20Constructor%3E(Base%3A%20TBase)%20%7B%0D%0A%20%20abstract%20class%20Inner1%20extends%20Base%20%7B%20%20%20%2F%2F%20Same%20as%20Outer1%0D%0A%20%20%20%20abstract%20same%3A%20number%3B%0D%0A%20%20%7D%0D%0A%20%20return%20Inner1%3B%0D%0A%7D%0D%0A%0D%0Afunction%20Mixin2%3CTBase%20extends%20Constructor%3E(Base%3A%20TBase)%20%7B%0D%0A%20%20abstract%20class%20Inner2%20extends%20Base%20%7B%20%20%20%2F%2F%20Same%20as%20Outer2%0D%0A%20%20%20%20abstract%20same%3A%20number%3B%0D%0A%20%20%7D%0D%0A%20%20return%20Inner2%3B%0D%0A%7D%0D%0A%0D%0Afunction%20rollUp%3CT%3E(mixins)%3A%20new%20(...args)%20%3D%3E%20T%20%7B%0D%0A%20%20return%20mixins.reduce((acc%2C%20mixin)%20%3D%3E%20mixin(acc)%2C%20class%20Seed%20%7B%7D)%3B%0D%0A%7D%0D%0A%0D%0Aclass%20Example%20extends%20rollUp%3COuter1%20%26%20Outer2%3E(%5BMixin1%2C%20Mixin2%5D)%20%7B%0D%0A%20%20%2F%2F%20Compiler%20will%20throw%20error%20when%20%22notSame%22%20is%20unimplemented%0D%0A%20%20%2F%2F%20Compiler%20DOES%20NOT%20throw%20error%20about%20non-implemented%20%22same%22%20member%0D%0A%7D | Bug,Help Wanted | low | Critical |
353,160,936 | TypeScript | Bad formatting adding multiple properties to start of object literal | **TypeScript Version:** As of #26588
**Code**
In `codeFixCannotFindModule_generateTypes_all.ts`:
```json
{
"compilerOptions": {
"baseUrl": ".",
"paths": { "*": ["types/*"] },
}
}
```
**Expected behavior:**
Closing brace of "compilerOptions" is indented.
**Actual behavior:**
Not indented. | Bug,Help Wanted,Domain: Formatter | low | Minor |
353,177,011 | godot | Going back in the Inspector while editing a GridMap node makes it and the previous node selected | <!-- Please search existing issues for potential duplicates before filing yours:
https://github.com/godotengine/godot/issues?q=is%3Aissue
-->
**Godot version:**
2db4942
**Issue description:**
Basically this:
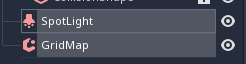 | bug,topic:editor,confirmed | low | Major |
353,194,067 | vue | transitionend event isn't fired on element inside <transition> when leaving | ### Version
2.5.17
### Reproduction link
[https://jsfiddle.net/guanzo/xcLk083u/1/](https://jsfiddle.net/guanzo/xcLk083u/1/)
### Steps to reproduce
1. Open reproduction demo in a non-Chrome browser.
2. Attach `transitionend` listener to the component root $el. (It can be on any element, doesn't matter)
3. Transition out a child element with `<transition>` and `v-if`.
4. Listener callback isn't fired.
### What is expected?
The transitionend event is fired in all browsers.
### What is actually happening?
The transitionend event is only fired in Chrome.
---
My use case does NOT allow me to use the template, therefore I cannot use the <transition> javascript hooks, such as `leave` and `afterLeave`. Regardless, this is inconsistent behavior and should be figured out. Is vue stopping the propagation of the event somehow?
For a complete context of the use case, read this issue: https://github.com/guanzo/vue-smooth-reflow/issues/3
<!-- generated by vue-issues. DO NOT REMOVE --> | transition | low | Minor |
353,200,426 | pytorch | [Feature Request] Crop op or ConvTranspose with output_shape | can you consider:
Add Crop op or ConvTranspose with output_shape...
Currently, there is no way to do a FCN Segment gladly... | caffe2 | low | Minor |
353,231,405 | go | cmd/go: provide some way to get the gofmt binary path | We can ask cmd/go for the path to cmd/compile:
```
$ go tool -n compile
/Users/josh/go/tip/pkg/tool/darwin_amd64/compile
```
There does not seem to be any way to ask cmd/go where the gofmt binary is.
```
$ go tool -n fmt
go tool: no such tool "fmt"
$ go fmt -n
can't load package: package .: found packages main (w.go) and p (x.go) in /Users/josh/go/tip/src
```
cc @rsc @bcmills
| NeedsDecision,GoCommand | low | Minor |
353,233,497 | go | cmd/compile: rename a bunch of things | Over time, I've noticed many things in the compiler that I'd like to rename, but I haven't because of the merge pain it causes and the review annoyance. I propose to use this issue to accumulate (and bikeshed) a list of renamings that we as a community want. Then a trusted contributor can do a single, automated bulk rename all in one commit.
I think this should happen shortly after the freeze starts. That gives everyone maximum opportunity to get all their CLs submitted. (Doing it early in the cycle is the worst idea, as it potentially breaks three months of private CL work.)
I'll start off the list with:
```
OPROC -> OGO
```
I'd also like new names for all of `Etop`, `Erv`, `Etype`, `Ecall`, `Efnstruct`, `Easgn`, and `Ecomplit`, but don't have good suggestions.
| compiler/runtime | medium | Critical |
353,273,662 | rust | Bad suggestion for overflowing hex literal in signed operation | Code:
```rust
pub fn flip_msb(a: i32) -> i32 {
a ^ 0x8000_0000
}
```
Diagnostic:
```
warning: literal out of range for i32
--> src/lib.rs:2:9
|
2 | a ^ 0x8000_0000
| ^^^^^^^^^^^
|
= note: #[warn(overflowing_literals)] on by default
= note: the literal `0x8000_0000` (decimal `2147483648`) does not fit into an `i32` and will become `-2147483648i32`
= help: consider using `u32` instead
```
In this case using `u32` is not an option (you could use a `u32` literal and cast to `i32`, but that's not ideal). A better suggestion in this case would be to use `-0x8000_0000` instead.
This issue is, I think, more relevant to hex (or other non-decimal) representations, as for example `format!("{:#x}", i32::MIN)` would produce `0x80000000`, which could lead someone to think that that is a valid warning-free `i32` literal. | C-enhancement,A-diagnostics,T-compiler | low | Major |
353,287,891 | vscode | Add a command that redos the last command that was executed | Please add a "repeat last command" command and keybinding, as sometimes found in other other apps, e.g. Microsoft Office.
I want to press a shortcut, and for it to repeat whatever the last command was, so I don't have to find it again in the menu or the <kbd>ctrl-shift-p</kbd> box.
This is especially useful when doing a lot of editing. | feature-request,keybindings | medium | Critical |
353,292,733 | rust | Support a TUI "live view" version of -Z self-profile. | Using [`tui`](https://docs.rs/tui) and by passing, say, the entire query stack to an "UI" thread 60 times per second, we can provide both a live view of the query stack, including when each entry started, *and* overall stats.
cc @nikomatsakis @michaelwoerister @wesleywiser @solson | C-enhancement,T-compiler,A-self-profile | low | Major |
353,306,119 | flutter | [iOS Suggestion] FlutterPluginRegistrar support remoteControlReceivedWithEvent | I am writing a plugin package which can play music by using AVPlayer.
I want to handle iPhone control in class FlutterPlugin, not in class AppDelegate.
I have called `[[UIApplication sharedApplication] beginReceivingRemoteControlEvents];` , but I can't listen the `remoteControlReceivedWithEvent ` in class FlutterPlugin. | platform-ios,engine,P3,a: plugins,team-ios,triaged-ios | low | Minor |
353,329,577 | kubernetes | Conflict error when create pod because resource quota version is old | <!-- This form is for bug reports and feature requests ONLY!
If you're looking for help check [Stack Overflow](https://stackoverflow.com/questions/tagged/kubernetes) and the [troubleshooting guide](https://kubernetes.io/docs/tasks/debug-application-cluster/troubleshooting/).
If the matter is security related, please disclose it privately via https://kubernetes.io/security/.
-->
**Is this a BUG REPORT or FEATURE REQUEST?**:
/kind bug
**What happened**:
In k8s.io/client-go/util/workqueue/queue.go
[https://github.com/kubernetes/client-go/blob/master/util/workqueue/queue.go](url)
1.
```
func (q *Type) Add(item interface{}) {
q.cond.L.Lock()
defer q.cond.L.Unlock()
if q.shuttingDown {
return
}
if q.dirty.has(item) {
return
}
q.metrics.add(item)
q.dirty.insert(item)
if q.processing.has(item) {
return
}
q.queue = append(q.queue, item)
q.cond.Signal()
}
```
```
func (q *Type) Get() (item interface{}, shutdown bool) {
q.cond.L.Lock()
defer q.cond.L.Unlock()
for len(q.queue) == 0 && !q.shuttingDown {
q.cond.Wait()
}
if len(q.queue) == 0 {
// We must be shutting down.
return nil, true
}
item, q.queue = q.queue[0], q.queue[1:]
q.metrics.get(item)
q.processing.insert(item)
q.dirty.delete(item)
return item, false
}
```
In add() function , we want to add the unique item in the queue.
In get() function, if more than 1 consumers , they will be waiting until producer put item into the queue.
If 10 consumers are waiting and 10 same items are put into the queue, the 10 items will be all consumed. Maybe we expect only **1** consumer to consume the same item because the item is reduplicated.
<br/>
2.
Everything is OK, but there is some problems in kube-controller-manager.
I use the example of resource_quota_controller:
[https://github.com/kubernetes/kubernetes/blob/master/pkg/controller/resourcequota/resource_quota_controller.go](url)
There are 6 workers are working.
```
for i := 0; i < workers; i++ {
go wait.Until(rq.worker(rq.queue), time.Second, stopCh)
go wait.Until(rq.worker(rq.missingUsageQueue), time.Second, stopCh)
}
```
```
func (rq *ResourceQuotaController) worker(queue workqueue.RateLimitingInterface) func() {
workFunc := func() bool {
key, quit := queue.Get()
if quit {
return true
}
defer queue.Done(key)
err := rq.syncHandler(key.(string))
if err == nil {
queue.Forget(key)
return false
}
utilruntime.HandleError(err)
queue.AddRateLimited(key)
return false
}
return func() {
for {
if quit := workFunc(); quit {
glog.Infof("resource quota controller worker shutting down")
return
}
}
}
}
```
When we update or delete a deployment, if there are 10 pod in this deployment, there are 10 same key(the namespace/name of quota) will be put into the queue. And the 6 workers are waked up and to deal with the same key.
Quota version maybe expires after re-calculation and the expired quota will update unsuccessfully because other workers have updated the quota.
There are too many conflict error will be logged.
```
Operation cannot be fulfilled on resourcequotas \"pod-quota\": the object has been modified; please apply your changes to the latest version and try again
```
<br/>
3.
Up to now, there is only warning and no error is happened.
But We maybe fail to create pod this time, the error info is :
```
{"kind":"Status","apiVersion":"v1","metadata":{},"status":"Failure","message":"Operation cannot be fulfilled on resourcequotas \"pod-quota\": the object has been modified; please apply your changes to the latest version and try again","reason":"Conflict","details":{"name":"pod-quota","kind":"resourcequotas"},"code":409}
```
Because the resource quota will calculate and update before create a pod. And if some error occurs, the function will retry 3 times. And if api-server doesn't update the quota successfully after 3 times, it will return back an error for user.
```
e.checkQuotas(quotas, admissionAttributes, 3)
func (e *quotaEvaluator) checkQuotas(quotas []api.ResourceQuota, admissionAttributes []*admissionWaiter, remainingRetries int) {
......
for i := range quotas {
newQuota := quotas[i]
// if this quota didn't have its status changed, skip it
if quota.Equals(originalQuotas[i].Status.Used, newQuota.Status.Used) {
continue
}
if err := e.quotaAccessor.UpdateQuotaStatus(&newQuota); err != nil {
updatedFailedQuotas = append(updatedFailedQuotas, newQuota)
lastErr = err
}
}
e.checkQuotas(quotasToCheck, admissionAttributes, remainingRetries-1)
}
```
**How to reproduce it (as minimally and precisely as possible)**:
1. create a resource quota , and create 50 pod by 1 deployment
2. delete the deployment and you can watch so many log like this:"please apply your changes to the latest version and try again"
**I hope that**:
1. reduce the conflict log and error by up the log level?
2. not wait but return if the queue is empty. or add an function like "peek()"?
3. add retry delay when error occurs in create pod period?
| kind/bug,sig/api-machinery,lifecycle/frozen | medium | Critical |
353,332,079 | pytorch | [Caffe2] Can't load pretrained model | If you have a question or would like help and support, please ask at our
[forums](https://discuss.pytorch.org/).
If you are submitting a feature request, please preface the title with [feature request].
If you are submitting a bug report, please fill in the following details.
## Issue description
I ran code from (this caffe2 tutorial - "Loading Pre-Trained Models")[https://caffe2.ai/docs/tutorial-loading-pre-trained-models.html] and code is failing with error
```
---------------------------------------------------------------------------
RuntimeError Traceback (most recent call last)
<ipython-input-14-314dd918183e> in <module>()
7
8 # Initialize the predictor from the input protobufs
----> 9 p = workspace.Predictor(init_net, predict_net)
10
11 # Run the net and return prediction
/opt/caffe2/caffe2/python/workspace.py in Predictor(init_net, predict_net)
169
170 def Predictor(init_net, predict_net):
--> 171 return C.Predictor(StringifyProto(init_net), StringifyProto(predict_net))
172
173
RuntimeError: [enforce fail at operator.cc:58] blob != nullptr. op Conv: Encountered a non-existing input blob: data
```
As i searched the web, I saw that some people add this line before net initialization to prevent this issue from occuring
```
workspace.FeedBlob("data", img)
```
But unfortunatelly, another error pops out
```---------------------------------------------------------------------------
RuntimeError Traceback (most recent call last)
<ipython-input-14-314dd918183e> in <module>()
7
8 # Initialize the predictor from the input protobufs
----> 9 p = workspace.Predictor(init_net, predict_net)
10
11 # Run the net and return prediction
/opt/caffe2/caffe2/python/workspace.py in Predictor(init_net, predict_net)
169
170 def Predictor(init_net, predict_net):
--> 171 return C.Predictor(StringifyProto(init_net), StringifyProto(predict_net))
172
173
RuntimeError: [enforce fail at operator.cc:58] blob != nullptr. op Conv: Encountered a non-existing input blob: conv1_w
```
It's most likely that Caffe2 initialization of `squeezenet` is corrupt. Any info how to fix this issue would be great.
I tried downloading `.pb` files both from amazon and github.
## Code example
Please try to provide a minimal example to repro the bug.
Error messages and stack traces are also helpful.
```workspace.FeedBlob("data", img)
with open(INIT_NET, "rb") as f:
init_net = f.read()
with open(PREDICT_NET, "rb") as f:
predict_net = f.read()
# Initialize the predictor from the input protobufs
p = workspace.Predictor(init_net, predict_net)
```
## System Info
Please copy and paste the output from our
[environment collection script](`)
(or fill out the checklist below manually).
You can get the script and run it with:
```
wget https://raw.githubusercontent.com/pytorch/pytorch/master/torch/utils/collect_env.py
# For security purposes, please check the contents of collect_env.py before running it.
python collect_env.py
```
- PyTorch or Caffe2: 0.8.1
- How you installed PyTorch (conda, pip, source): Docker image of caffe2 from NVCR.io
- OS: Linux 0cf485e1c286 4.15.0-29-generic #31-Ubuntu SMP Tue Jul 17 15:39:52 UTC 2018 x86_64 x86_64 x86_64 GNU/Linux
- Python version: Python 3.5.2
- CUDA/cuDNN version: V9.0.176
- GPU models and configuration: GeForce GTX 1070 | caffe2 | low | Critical |
353,352,859 | rust | const fold .repeat() of same-char string literals | ````rustc 1.30.0-nightly (33b923fd4 2018-08-18)````
````rust
pub fn bla() -> String {
"aaa".to_string()
}
````
this currently generates
````asm
example::bla:
push rbx
mov rbx, rdi
lea rsi, [rip + .L__unnamed_1]
mov edx, 3
call <alloc::string::String as core::convert::From<&'a str>>::from@PLT
mov rax, rbx
pop rbx
ret
.L__unnamed_1:
.zero 3,97
````
now if we used ````.repeat(3)```` (this only applies for strings consisting only of one character sort),
````rust
pub fn bla() -> String {
"aaa".to_string().repeat(3)
}
````
we get this monster:
````asm
core::ptr::drop_in_place:
mov rsi, qword ptr [rdi + 8]
test rsi, rsi
je .LBB0_1
mov rdi, qword ptr [rdi]
mov edx, 1
jmp __rust_dealloc@PLT
.LBB0_1:
ret
example::bla:
push r14
push rbx
sub rsp, 24
mov r14, rdi
lea rsi, [rip + .L__unnamed_1]
mov rdi, rsp
mov edx, 3
call <alloc::string::String as core::convert::From<&'a str>>::from@PLT
mov rbx, qword ptr [rsp]
mov rdx, qword ptr [rsp + 16]
mov ecx, 3
mov rdi, r14
mov rsi, rbx
call alloc::str::<impl str>::repeat@PLT
mov rsi, qword ptr [rsp + 8]
test rsi, rsi
je .LBB1_3
mov edx, 1
mov rdi, rbx
call __rust_dealloc@PLT
.LBB1_3:
mov rax, r14
add rsp, 24
pop rbx
pop r14
ret
mov rbx, rax
mov rdi, rsp
call core::ptr::drop_in_place
mov rdi, rbx
call _Unwind_Resume@PLT
ud2
.L__unnamed_1:
.zero 3,97
````
although we could probably just have
````asm
example::bla:
push rbx
mov rbx, rdi
lea rsi, [rip + .L__unnamed_1]
mov edx, 9
call <alloc::string::String as core::convert::From<&'a str>>::from@PLT
mov rax, rbx
pop rbx
ret
.L__unnamed_1:
.zero 9,97
````
(diff to the first version)
````diff
@@ -2,11 +2,11 @@
push rbx
mov rbx, rdi
lea rsi, [rip + .L__unnamed_1]
- mov edx, 3
+ mov edx, 9
call <alloc::string::String as core::convert::From<&'a str>>::from@PLT
mov rax, rbx
pop rbx
ret
.L__unnamed_1:
- .zero 3,97
+ .zero 9,97
```` | I-slow,C-enhancement,A-codegen,T-compiler | low | Minor |
353,370,648 | vscode | Allow <super> as a keybinding option in linux | I am using VSCode on linux (fedora) and I want to bind the *super* key to:
"editor.multiCursorModifier": "super"
but currently, the only options are ctrlCmd and alt (which doesn't work because it toggles menu navigation).
I don't want to change it to ctrl+click because it's handy for links and function definitions, but multi-cursor editing is also very useful, and I can't make both happen with my keybinding options.
| feature-request,editor-multicursor | low | Major |
353,373,626 | TypeScript | Overriding generic method inconsistently errors | <!-- 🚨 STOP 🚨 𝗦𝗧𝗢𝗣 🚨 𝑺𝑻𝑶𝑷 🚨
Half of all issues filed here are duplicates, answered in the FAQ, or not appropriate for the bug tracker. Even if you think you've found a *bug*, please read the FAQ first, especially the Common "Bugs" That Aren't Bugs section!
Please help us by doing the following steps before logging an issue:
* Search: https://github.com/Microsoft/TypeScript/search?type=Issues
* Read the FAQ: https://github.com/Microsoft/TypeScript/wiki/FAQ
Please fill in the *entire* template below.
-->
<!-- Please try to reproduce the issue with `typescript@next`. It may have already been fixed. -->
**TypeScript Version:** 3.1.0-dev.201xxxxx
<!-- Search terms you tried before logging this (so others can find this issue more easily) -->
**Search Terms:** override generic
**Code**
```ts
interface Foo {
method<T>(): T;
}
// Error: Interface 'Bar<T>' incorrectly extends interface 'Foo'.
interface Bar<T extends Node> extends Foo {
method<T2 extends T>(): T2;
}
// Uncommenting this suddenly fixes the error
// interface Foo {
// method<T>(): T;
// }
```
Full error message:
```
Interface 'Bar<T>' incorrectly extends interface 'Foo'.
Types of property 'method' are incompatible.
Type '<T2 extends T>() => T2' is not assignable to type '<T>() => T'.
Type 'T' is not assignable to type 'T'. Two different types with this name exist, but they are unrelated.
Type 'Node' is not assignable to type 'T'.
```
**Expected behavior:** The error should always appear, or always not appear.
**Actual behavior:** A partial interface mysteriously removes the error
**Playground Link:** https://www.typescriptlang.org/play/#src=interface%20Foo%20%7B%0D%0A%20%20%20%20method%3CT%3E()%3A%20T%3B%0D%0A%7D%0D%0A%0D%0Ainterface%20Bar%3CT%20extends%20Node%3E%20extends%20Foo%20%7B%0D%0A%20%20%20%20method%3CT2%20extends%20T%3E()%3A%20T2%3B%0D%0A%7D%0D%0A%0D%0A%2F%2F%20Uncommenting%20this%20suddenly%20fixes%20the%20error%0D%0A%2F%2F%20interface%20Foo%20%7B%0D%0A%2F%2F%20%20%20%20%20method%3CT%3E()%3A%20T%3B%0D%0A%2F%2F%20%7D
**Related Issues:** #23960
| Bug,Help Wanted | low | Critical |
353,376,853 | go | encoding/json: clarify merge semantics of Unmarshal | Please answer these questions before submitting your issue. Thanks!
### What version of Go are you using (`go version`)?
Go playground
### Does this issue reproduce with the latest release?
Yes
### What operating system and processor architecture are you using (`go env`)?
Go playground
### What did you do?
https://play.golang.org/p/QM3FKwmR9h1
### What did you expect to see?
```
{john}
{smith}
```
### What did you see instead?
```
{john }
{john smith}
```
### In short...
The Unmarshal documentation says:
> Unmarshal parses the JSON-encoded data and stores the result in the value pointed to by v.
~ https://golang.org/pkg/encoding/json/#Unmarshal
I assumed that after Unmarshal returns, the struct would *exactly* match the provided JSON. I found this quite surprising and I think the documentation should mention that "Fields that are not present in the JSON document will retain their original values."
The existing functionality does give one an elegant way to set default values by having Unmarshal only update those fields which are present in the JSON document. I do not dispute that it is useful. Still, I would like to see the documentation mention this behaviour explicitly. | Documentation,NeedsFix | low | Minor |
353,419,098 | svelte | Break out individual compilers | As frequenters of the Discord server will know, I'm become obsessed lately with the idea of [compiling Svelte components to WebGL code](https://github.com/Rich-Harris/svelte-gl) (not for UI, but for building 3D/VR scenes — the sort of thing you'd traditionally use Three.js or A-Frame for). The easiest way to try this out would be to create a new compile target inside Svelte itself, but that would be somewhat disruptive for something that *may* turn out to be completely impossible.
An alternative, which I think is worth doing *anyway* as it would be good for the codebase, is to break out different compilers into their own packages. So instead of every `Node` subclass implementing `build` (for the `dom` target) and `ssr` (for the `ssr` target), the program generated in the initial phase would be handed off to a compiler, which would return something like a `{ code, map }` object (or otherwise something that can be easily turned into one, if that makes more sense in terms of code reuse etc).
So we'd have `@sveltejs/compile-dom`, `@sveltejs/compile-ssr` and (eventually) `@sveltejs/compile-gl`, each implementing the same interface. In time we could even add to that list — `@sveltejs/compile-native`, `@sveltejs/compile-tty` and so on.
In all likelihood this would need to be accompanied by separate runtime libraries for the different targets — `@sveltejs/lib-dom`, `@sveltejs/lib-ssr`, `@sveltejs/lib-common` (which `dom` and `ssr` would re-export from).
Aside from all the speculative VR stuff, I think this would probably make the codebase less intimidating for new contributors, and it would be good discipline. (The `svelte` package would still bundle everything, we wouldn't have a nightmarish situation with peer dependencies getting out of sync.)
Any thoughts? | compiler | medium | Major |
353,434,451 | go | x/tools/go/ssa/interp: panic: no code for function: (reflect.Value).MapRange | These brittle tests break again:
```
Input: boundmeth.go
PASS
Input: complit.go
panic: no code for function: (reflect.Value).MapRange
FAIL
To trace execution, run:
% go build golang.org/x/tools/cmd/ssadump && ./ssadump -build=C -test -run --interp=T complit.go
Input: coverage.go
PASS
Input: defer.go
PASS
Input: fieldprom.go
PASS
Input: ifaceconv.go
PASS
Input: ifaceprom.go
PASS
Input: initorder.go
PASS
Input: methprom.go
PASS
Input: mrvchain.go
PASS
Input: range.go
PASS
Input: recover.go
PASS
Input: reflect.go
PASS
Input: static.go
PASS
Input: callstack.go
PASS
The following tests failed:
complit.go
--- FAIL: TestTestdataFiles (24.76s)
interp_test.go:235: interp.Interpret([testdata/complit.go]) failed: exit code was 2
FAIL
FAIL golang.org/x/tools/go/ssa/interp 25.796s
```
| Testing,NeedsFix,Tools | low | Critical |
353,451,646 | flutter | Support any gesture recognizers for embedded platform views | Right now the `gestureRecognizers` parameter in AndroidView is a list of `OneSequenceGestureRecognizer`s as currently only `OneSequenceGestureRecognizer`s support gesture arena teams.
Ideally `gestureRecognizers` should be a list of `GestureRecognizer`s. | c: new feature,framework,engine,f: gestures,a: platform-views,c: proposal,P2,team-engine,triaged-engine | low | Major |
353,497,986 | go | encoding/json: no way to preserve the order of map keys | The encoding/json library apparently offers no way to decode data into a interface{} without scrambling the order of the keys in objects. Generally this isn't a correctness issue (per the spec the order isn't important), but it is super annoying to humans who may look at JSON data before and after it's been processed by a go program.
The most popular yaml library solves the problem like this: https://godoc.org/gopkg.in/yaml.v2#MapSlice | NeedsDecision,FeatureRequest | high | Critical |
353,567,461 | TypeScript | In JS, module.exports assignment doesn't make types available | **Code**
```js
// in a javascript file mod1.js:
class C {
prop = 0;
}
module.exports = { C }
```
```ts
// in a typescript file test.ts
declare var yyy: import("./mod1").C
```
**Expected behavior:**
No error, and `yyy: C`
**Actual behavior:**
Error, namespace '"src/test/mod1".export=' has no exported member 'C'.
module.exports-assigned object literals should be marked with SymbolFlags.Namespace, or perhaps getTypeFromImportTypeNode needs to know about non-namespace containers. It probably also needs to check members instead of exports, since that's where object literals store their members. | Bug,Domain: JavaScript | low | Critical |
353,568,315 | node | Add testcases for all documented safeguards | _To clarify: I am not blaming anyone. I view this as a problem of the process that we used, the aim of this issue is to seek how we can actually improve things. I myself LGTMd the change that introduced the initial safeguard without a testcase._
This is a post-mortem of https://github.com/nodejs-private/security/issues/202 (see 40a7beeddac9b9ec9ef5b49157daaf8470648b08).
That itself was a minor issue which is very unlikely to be exploitable (more unlikely than the second one fixed in the same release), but it revealed what I think is a process problem.
That issue was introduced in #18790 which removed the corresponding safeguard from the code while optimizing performance. The safeguard was initially introduced in #4682, and was documented in the code — that didn't save it from being removed:
> This prevents accidentally sending in a number that would be interpretted as a start offset.
My opinion is that a testcase should have been introduced at the same time when the comment was. Or, perhaps, even _instead_ of the comment — the comment turned out to be not effective.
So, the proposal is to:
1. Collect a list of similar comments in the codebase that warn against changing some code or explain why is it needed — that hints that the common usecase might work with that code removed, but doing so is expected to break some rare usecase or cause a potential security issue.
2. Try removing/modifying the safegaurds and see if any tests break, make a list of safeguards that do not break tests when are tampered with.
3. Add missing tests.
4. Update to try spotting such things (i.e. untested expectations) in the future while reviewing PRs. Should be fairly easy to spot things that were worth a comment in the code and to question _if those are tested_?
There might be none — but I would prefer if we re-checked that.
Side note: code coverage is not an effective measure to collect the data here or prevent the issue.
/cc @nodejs/security-wg | help wanted,test,security | low | Major |
353,575,496 | go | cmd/compile: understand Go vs assembly rc4 results | Tracking bug so somebody (@randall77?) looks into why we got totally opposite performance numbers on different Intel CPUs when we deleted the crypto/rc4 code's assembly in "favor" of the Go version in 30eda6715c6578de2086f03df36c4a8def838ec2 (https://golang.org/cl/130397):
```
name old time/op new time/op delta
RC4_128-4 256ns ± 0% 196ns ± 0% -23.78% (p=0.029 n=4+4)
RC4_1K-4 2.38µs ± 0% 1.54µs ± 0% -35.22% (p=0.029 n=4+4)
RC4_8K-4 19.4µs ± 1% 12.0µs ± 0% -38.35% (p=0.029 n=4+4)
name old speed new speed delta
RC4_128-4 498MB/s ± 0% 654MB/s ± 0% +31.12% (p=0.029 n=4+4)
RC4_1K-4 431MB/s ± 0% 665MB/s ± 0% +54.34% (p=0.029 n=4+4)
RC4_8K-4 418MB/s ± 1% 677MB/s ± 0% +62.18% (p=0.029 n=4+4)
vendor_id : GenuineIntel
cpu family : 6
model : 142
model name : Intel(R) Core(TM) i5-7Y54 CPU @ 1.20GHz
stepping : 9
microcode : 0x84
cpu MHz : 800.036
cache size : 4096 KB
name old time/op new time/op delta
RC4_128-4 235ns ± 1% 431ns ± 0% +83.00% (p=0.000 n=10+10)
RC4_1K-4 1.74µs ± 0% 3.41µs ± 0% +96.74% (p=0.000 n=10+10)
RC4_8K-4 13.6µs ± 1% 26.8µs ± 0% +97.58% (p=0.000 n=10+9)
name old speed new speed delta
RC4_128-4 543MB/s ± 0% 297MB/s ± 1% -45.29% (p=0.000 n=10+10)
RC4_1K-4 590MB/s ± 0% 300MB/s ± 0% -49.16% (p=0.000 n=10+10)
RC4_8K-4 596MB/s ± 1% 302MB/s ± 0% -49.39% (p=0.000 n=10+9)
vendor_id : GenuineIntel
cpu family : 6
model : 63
model name : Intel(R) Xeon(R) CPU @ 2.30GHz
stepping : 0
microcode : 0x1
cpu MHz : 2300.000
cache size : 46080 KB
```
Super mysterious, so we might want to understand it enough to decide whether we care and whether there's something the compiler might do better for more CPUs.
Maybe the benchmarks or benchstat are wrong? But then that'd be its own interesting bug.
/cc @josharian @aead @FiloSottile | Performance,NeedsInvestigation,compiler/runtime | low | Critical |
353,600,855 | go | cmd/go: using go test, goroutine stack dump sometimes not emitted on SIGQUIT | ### What version of Go are you using (`go version`)?
Tried `go version go1.10.3 darwin/amd64`, `go version go1.11rc1 darwin/amd64`, and `go version devel +6e76aeba0b Thu Aug 23 20:27:47 2018 +0000 darwin/amd64`.
### What did you do?
I had a test that appeared to be stuck in a blocking operation, and I pressed `^\` to send SIGQUIT, but I didn't receive any output. Then when I ran the test again using `go test -v`, sending SIGQUIT produced the goroutine stacks I expected to see.
Here's a sample test I'm using in the following output:
```go
package main
import (
"testing"
"time"
)
func TestSleep(t *testing.T) {
time.Sleep(time.Minute)
}
```
Running just `go test .` doesn't produce output on SIGQUIT, but I do see output with `go test -v .`.
```
$ /usr/local/Cellar/go/1.10.3/bin/go test .
^\
$ /usr/local/Cellar/go/1.10.3/bin/go test -v .
=== RUN TestSleep
^\SIGQUIT: quit
PC=0x105508b m=0 sigcode=0
goroutine 0 [idle]:
...
FAIL _/tmp/b 2.084s
$ gotip test .
^\
$ gotip test -v .
=== RUN TestSleep
^\SIGQUIT: quit
PC=0x7fff706a6a1e m=0 sigcode=0
goroutine 0 [idle]:
...
FAIL _/tmp/b 1.859s
```
If I change the file to include a test that calls t.Log, then I seem to be able to get a stack dump with tip, but not with 1.10 or 1.11rc1:
```
$ cat sleep_test.go
package main
import (
"testing"
"time"
)
func TestPass(t *testing.T) {
t.Logf("ok")
}
func TestSleep(t *testing.T) {
time.Sleep(time.Minute)
}
$ /usr/local/Cellar/go/1.10.3/bin/go test .
^\
$ go test .
^\
$ gotip test .
^\SIGQUIT: quit
PC=0x7fff706a6a1e m=0 sigcode=0
goroutine 0 [idle]:
...
FAIL _/tmp/b 6.311s
```
If I comment out the t.Logf call, but keep `TestPass`, then gotip no longer produces a stack dump on SIGQUIT. | NeedsInvestigation,GoCommand | low | Major |
353,642,067 | opencv | cvLoadImage on iOS |
##### System information (version)
- OpenCV => 3.4.1
- Operating System / Platform =>Mac 10.13.4
- Compiler => Xcode
##### Detailed description
I would like to load a png image with `cvLoadImage`, it return nil unfortunately, but a 'jpg' type is ok.
##### Steps to reproduce
```
NSString *phonePath = [[NSBundle mainBundle] pathForResource:@"phone" ofType:@"png"];
IplImage *src = cvLoadImage([phonePath UTF8String]);//nil
``` | platform: ios/osx,incomplete | low | Minor |
353,645,032 | rust | #[repr(u16)] enum doesn't pack fields as expected | The following code:
```rust
#[repr(u16)] enum Foo { A, B(u32, u16) }
println!("{}", std::mem::size_of::<Foo>());
```
currently outputs 12, but `Foo` really only needs to take 8 bytes.
There are several alternatives which can correctly be packed into 8 bytes:
```rust
#[repr(u8)] enum Foo { A, B(u32, u16) }
```
```rust
#[repr(u16)] enum Foo { A, B(u16, u32) }
```
I see no reason why the first enum couldn't be packed into 8 bytes. | A-type-system,A-codegen,T-compiler,C-bug,T-types,A-repr,A-enum | low | Minor |
353,670,783 | rust | Remove all definition names from the HIR. | If it's in `tcx.def_key(def_id)` that's a better source of truth and available cross-crate.
Only reason I could see for keeping names is to point at their span
* we could also keep that separately, even cross-crate
* we might want something that captures the "name introduction form" span
* e.g. the span of `fn name` or `const NAME`, not just the name
* sometimes we use the span of an entire item and it looks pretty bad
* we could also refer to things that way in human-facing printing
* e.g. "`fn fmt` in `impl fmt::Debug for Foo`" instead of `<Foo as fmt::Debug>::foo`
* or `(impl fmt::Debug for Foo)::fn foo`
cc @petrochenkov @michaelwoerister @nikomatsakis | C-cleanup,T-compiler | low | Critical |
353,711,250 | material-ui | [Checkbox] Add checked animation | The [Checkbox](https://material-ui.com/api/checkbox/) component is missing the animation for the icon when toggling it. This makes checking the checkbox look bit cluncky, especially when toggling it from the label.
In material design demo you can clearly see the icon animated when you toggle it: https://material-components.github.io/material-components-web-catalog/#/component/checkbox
Compare it to the current implementation in material-ui. See especially how it looks when toggling it by clicking the label.
https://material-ui.com/components/checkboxes/
Even in v0 has some kind of animation, which in my opinion makes it look better than the current version https://v0.material-ui.com/#/components/checkbox
<!-- Checked checkbox should look like this: [x] -->
- [x] This is a v1.x issue. <!-- (v0.x is no longer maintained) -->
- [x] I have searched the [issues](https://github.com/mui-org/material-ui/issues) of this repository and believe that this is not a duplicate.
Edit: Updated outdated link | new feature,component: checkbox | medium | Critical |
353,747,426 | flutter | Animation lag when navigating to screen with `TextField` with `autoFocus` set to `true` on iOS | This effects only `iOS`. tested on `iPhone 6` and `iPad (3rd gen)`
##Demo
[https://drive.google.com/file/d/1LPMdNMf6z6yYBCYM3JMegMx-SQw0vGUs/view?usp=sharing](https://drive.google.com/file/d/1LPMdNMf6z6yYBCYM3JMegMx-SQw0vGUs/view?usp=sharing)
## Steps to Reproduce
1. set `autoFocus = true` on first `TextField` in `text_form_field_demo.dart` of Flutter Gallery.
2. Run the gallery in release mode and navigate to Material -> Text Fields
3. observe the lag.
if `autoFocus = true` is not set, then navigation animation is smooth. this seems to happen when the keyboard pops up.
```
[✓] Flutter (Channel dev, v0.5.8, on Mac OS X 10.13.2 17C88, locale en-IN)
• Flutter version 0.5.8 at /Users/hari/development/flutter
• Framework revision e4b989bf3d (2 weeks ago), 2018-08-09 09:45:44 -0700
• Engine revision 3777931801
• Dart version 2.0.0-dev.69.5.flutter-eab492385c
[✓] Android toolchain - develop for Android devices (Android SDK 27.0.3)
• Android SDK at /Users/hari/Library/Android/sdk
• Android NDK location not configured (optional; useful for native profiling support)
• Platform android-27, build-tools 27.0.3
• Java binary at: /Applications/Android Studio.app/Contents/jre/jdk/Contents/Home/bin/java
• Java version OpenJDK Runtime Environment (build 1.8.0_152-release-1024-b01)
• All Android licenses accepted.
[✓] iOS toolchain - develop for iOS devices (Xcode 9.4.1)
• Xcode at /Applications/Xcode.app/Contents/Developer
• Xcode 9.4.1, Build version 9F2000
• ios-deploy 1.9.2
• CocoaPods version 1.5.3
[✓] Android Studio (version 3.1)
• Android Studio at /Applications/Android Studio.app/Contents
• Flutter plugin version 27.1.1
• Dart plugin version 173.4700
• Java version OpenJDK Runtime Environment (build 1.8.0_152-release-1024-b01)
[✓] VS Code (version 1.26.1)
• VS Code at /Applications/Visual Studio Code.app/Contents
• Flutter extension version 2.17.1
[✓] Connected devices (1 available)
• iPad • 4517c71cd57462f4d77e237e4ead89f44ce99fa1 • ios • iOS 10.3.3
• No issues found!
```
| a: text input,framework,a: animation,c: performance,f: focus,has reproducible steps,P2,found in release: 3.3,found in release: 3.7,team-framework,triaged-framework | low | Critical |
353,762,895 | rust | Tracking issue for eRFC 2497, "if- and while-let-chains, take 2" | This is a tracking issue for the eRFC *"if- and while-let-chains, take 2"* (rust-lang/rfcs#2497).
For the tracking issue for the immediate edition changes, see #53668.
**Steps:**
- [x] Implement the RFC (cc @rust-lang/compiler -- can anyone write up mentoring instructions?)
- [ ] Adjust documentation ([see instructions on forge][doc-guide])
- [ ] Formatting for new syntax has been added to the [Style Guide] ([nightly-style-procedure])
- [ ] resolve expr fragment specifier issue ([#86730](https://github.com/rust-lang/rust/issues/86730))
- [x] Initial stabilization PR: #94927, reverted in #100538
- [ ] Second stabilization PR: https://github.com/rust-lang/rust/pull/132833
[stabilization-guide]: https://forge.rust-lang.org/stabilization-guide.html
[doc-guide]: https://forge.rust-lang.org/stabilization-guide.html#updating-documentation
[nightly-style-procedure]: https://github.com/rust-lang/style-team/blob/master/nightly-style-procedure.md
[Style Guide]: https://github.com/rust-lang/rust/tree/master/src/doc/style-guide
**Unresolved questions:**
- [x] [The final syntax](https://github.com/rust-lang/rfcs/blob/master/text/2497-if-let-chains.md#the-final-syntax)
- This would benefit from a design note summarizing previous discussions on `let` as a boolean expression, and possibilities of `is` syntax.
- [x] [Should temporary and irrefutable lets without patterns be allowed in some form?](https://github.com/rust-lang/rfcs/blob/master/text/2497-if-let-chains.md#irrefutable-let-bindings-after-the-first-refutable-binding)
- [ ] [Chained `if let`s inside `match` arms](https://github.com/rust-lang/rfcs/blob/master/text/2497-if-let-chains.md#chained-if-lets-inside-match-arms)
**Collected issues:**
- [x] https://github.com/rust-lang/rust/issues/60336
- [x] https://github.com/rust-lang/rust/issues/60707
- [x] https://github.com/rust-lang/rust/issues/60254
- [x] https://github.com/rust-lang/rust/issues/60571
- [x] https://github.com/rust-lang/rust/issues/44990
**Implementation history:**
- https://github.com/rust-lang/rust/pull/59290
- https://github.com/rust-lang/rust/pull/59439
- https://github.com/rust-lang/rust/pull/60225
- https://github.com/rust-lang/rust/pull/59288
- https://github.com/rust-lang/rust/pull/60861
- https://github.com/rust-lang/rust/pull/61988
- https://github.com/rust-lang/rust/pull/79328
- https://github.com/rust-lang/rust/pull/80357
- https://github.com/rust-lang/rust/pull/82308
- https://github.com/rust-lang/rust/pull/88642
- https://github.com/rust-lang/rust/pull/93086
- https://github.com/rust-lang/rust/pull/93213
- https://github.com/rust-lang/rust/pull/97295
- https://github.com/rust-lang/rust/pull/94754
- https://github.com/rust-lang/rust/pull/94974
- https://github.com/rust-lang/rust/pull/95008
- https://github.com/rust-lang/rust/pull/95314
**Unresolved problems**
- [ ] Can we be confident that the implementation is correct and well tested?
<!-- TRIAGEBOT_START -->
<!-- TRIAGEBOT_ASSIGN_START -->
<!-- TRIAGEBOT_ASSIGN_DATA_START$${"user":"c410-f3r"}$$TRIAGEBOT_ASSIGN_DATA_END -->
<!-- TRIAGEBOT_ASSIGN_END -->
<!-- TRIAGEBOT_END --> | B-RFC-approved,T-lang,C-tracking-issue,F-let_chains,S-tracking-ready-to-stabilize | high | Critical |
353,768,461 | flutter | VideoPlayer plugin should make sure that resources are released when FlutterView is destroyed | This is related to https://github.com/flutter/flutter/issues/19206 and https://github.com/flutter/flutter/issues/19358.
`VideoPlayer` plugin on Android allocates a platform `MediaPlayer` object for each `VideoPlayer` plugin in the widget tree. `MediaPlayer` objects [are destroyed](https://github.com/flutter/plugins/blob/master/packages/video_player/android/src/main/java/io/flutter/plugins/videoplayer/VideoPlayerPlugin.java#L225) when corresponding Flutter widgets go away. But if a whole `FlutterView` is destroyed, `dispose` methods don't run, so `MediaPlayer` instances are not released. This leads to leaks and also crashes (since the player continues to provide frames for non-existent surfaces; internal bug: b/112692333).
IMO, `VideoPlayer` should rely on normal disposing for the final cleanup and just assert that by the time the view is destroyed all resources are released. This would rely on widgets disposal methods to be called, which is tracked in https://github.com/flutter/flutter/issues/19358.
As a workaround, `VideoPlayer` should subscribe with a `onViewDestroyListener` and explicitly release all resources when the owner `FlutterView` goes away. | c: performance,p: video_player,package,team-ecosystem,P3,triaged-ecosystem | low | Critical |
353,771,576 | TypeScript | Intellisense for generic parameterized string literal function arguments not working | _From @njgraf512 on July 31, 2018 22:5_
<!-- Please search existing issues to avoid creating duplicates. -->
<!-- Also please test using the latest insiders build to make sure your issue has not already been fixed: https://code.visualstudio.com/insiders/ -->
<!-- Use Help > Report Issue to prefill these. -->
- VSCode Version: 1.26.0-insider
- OS Version: MacOS 10.12.6
Steps to Reproduce:
1. Enter the following into a TS file:
```ts
const myObj = {
foo: 'Foo',
bar: 'Bar',
}
function myFunc<T>(obj: T, key: keyof T) {
return obj[key];
}
myFunc(myObj, 'f')
```
2. Observe that once you begin typing the `f` of string literal `foo` for the function's second argument, the tooltip switches from suggesting `"foo" | "bar"` to `never`:
**Good:**
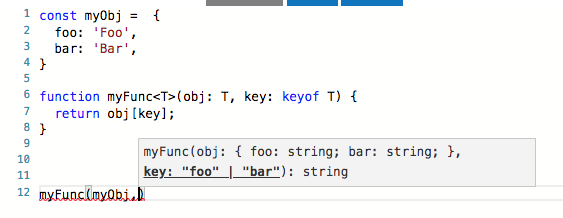
**Bad:**
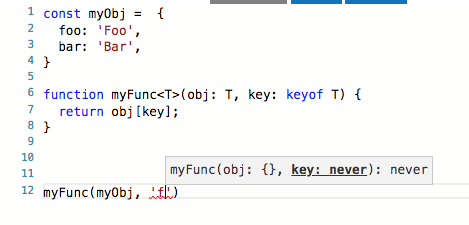
3. Also, triggering intellisense with <kbd>control</kbd>+<kbd>space</kbd> does not suggest `foo` or `bar`:
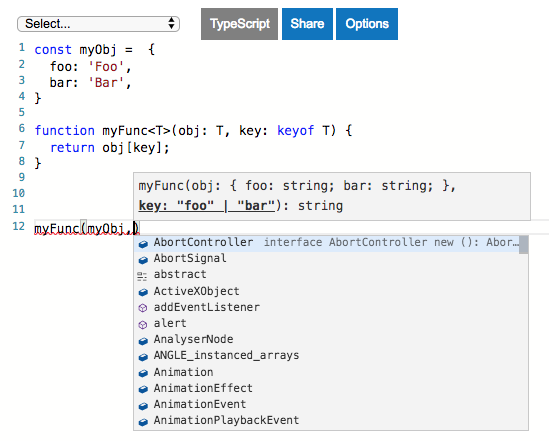
<!-- Launch with `code --disable-extensions` to check. -->
Does this issue occur when all extensions are disabled?: Yes/No
No
_Copied from original issue: Microsoft/vscode#55530_ | Suggestion,Domain: Completion Lists,VS Code Tracked,Experience Enhancement | low | Minor |
353,782,503 | rust | Fabricate hangs while building rust for raspbian on arm64 host. | We do a lot of package builds for raspbian in a raspbian chroot in a debian armhf container on an arm64 host, we use this host because it's fast.
Unfortunately recent versions of rustc can no longer be built in this environment, a program called "fabricate" hangs with 200% CPU usage. IIRC this has been happening since 1.25, currently I am building 1.27 with 1.26 (I will try again with 1.28 once I have 1.27 built).
running: "/<<BUILDDIR>>/rustc-1.27.2+dfsg1/build/arm-unknown-linux-gnueabihf/stage0-tools-bin/fabricate" "generate" "--product-name=Rust-Documentation" "--rel-manifest-dir=rustlib" "--success-message=Rust-documentation-is-installed." "--image-dir" "/<<BUILDDIR>>/rustc-1.27.2+dfsg1/build/tmp/dist/rust-docs-1.27.2-arm-unknown-linux-gnueabihf-image" "--work-dir" "/<<BUILDDIR>>/rustc-1.27.2+dfsg1/build/tmp/dist" "--output-dir" "/<<BUILDDIR>>/rustc-1.27.2+dfsg1/build/dist" "--package-name=rust-docs-1.27.2-arm-unknown-linux-gnueabihf" "--component-name=rust-docs" "--legacy-manifest-dirs=rustlib,cargo" "--bulk-dirs=share/doc/rust/html"
(the <<BUILDDIR>> shown in the log is a substitution made by a build log filter, the actual command has the real paths in it).
Attempts to debug/workaround this issue on the box in question have failed, my only soloution so-far has been to do the rust builds on a different box (which works but is a pain with my workflow).
To help me debug I would appreciate answers to the following questions.
1. What is fabricate and where can I find documentation for it? googling for "rust fabricate" or even "rust language fabricate" has proved futile.
2. How can I run commands from the rust build process outside the build process (e.g. in a debugger), in my experiance trying to re-run the commands shown by the build process inevitablly results in unrelated errors. Are there special environment variables in-play? if so how do I find out what those environment variables should be.
3. Is there a way to disable paralellism in fabricate?
| O-Arm,O-AArch64 | low | Critical |
353,895,620 | go | net: clarify net.Conn concurrency requirement | From the net.Conn docs:
> Multiple goroutines may invoke methods on a Conn simultaneously.
I'm not sure if this should be interpreted as *different* methods can be called concurrently, or as the same method can be called concurrently.
I suspect, judging from x/net/nettest's ConcurrentMethods and from most implementations, that it's the former. If so, should we change the docs to include "may invoke *distinct* methods"? | Testing,Documentation,help wanted,NeedsInvestigation | medium | Major |
353,927,343 | svelte | Slotted content for Web Component nested inside of a Svelte component is not applied to the Web Component | Given a Web Component which expects slotted children, which itself is nested inside of a Svelte component, as in the following:
```
<SvelteComponent>
<my-custom-element>
<div slot="slot1">my-custom-element content</div>
<div slot="slot2">more my-custom-element content</div>
</my-custom-element>
</SvelteComponent>
```
...the slotted children are not applied to the Web Component.
I have reproduced the issue here (just run ```npm install``` and ```npm run build``` and then open ```dist/index.html``` in your browser):
https://github.com/therealnicksaunders/svelte/tree/issue/slotted-content
In a more complicated example, this actually produces an error which suggests that a slotted child is being applied to the Svelte component instead of the Web Component in which it is nested. We have this Svelte-generated code:
```
(0, _shared.append)(pagecontent._slotted.header, div);
```
The referenced div is nested inside of a Web Component; its "slot" attribute of "header" corresponds to a slot inside of that Web Component rather than the PageContent Svelte component (which has no named slots).
Hopefully I have provided enough info here... Please let me know if I can clarify anything.
Thanks in advance for your help looking into it! | awaiting submitter,custom element | medium | Critical |
353,959,082 | go | cmd/go: -exec prevents test caching | ### What version of Go are you using (`go version`)?
go version go1.10.3 freebsd/amd64
### Does this issue reproduce with the latest release?
Yes
### What operating system and processor architecture are you using (`go env`)?
freebsd/amd64
### What did you do?
go test -exec wrap1.sh ./...
go test -exec wrap1.sh ./..
### What did you expect to see?
tests should be cached on the second attempt
### What did you see instead?
full tests ran
The reason for this is the following line: https://github.com/golang/go/blob/master/src/cmd/go/internal/test/test.go#L1106
```golang
execCmd := work.FindExecCmd()
if !c.disableCache && len(execCmd) == 0 {
testlogArg = []string{"-test.testlogfile=" + a.Objdir + "testlog.txt"}
}
```
While the documented set of 'cacheable' test flags don't include -exec it seems like this should be supported.
While looking into this issue I also noticed this specific criteria is not mentioned when ```GODEBUG``` includes ```gocachetest=1``` so at the least is should be output e.g.
```golang
execCmd := work.FindExecCmd()
if len(execCmd) != 0 {
fmt.Fprintf(os.Stderr, "testcache: caching disabled for cmd: %q\n", execCmd)
} else if !c.disableCache {
testlogArg = []string{"-test.testlogfile=" + a.Objdir + "testlog.txt"}
}
```
With more investigation I also identified that tryCacheWithID actually includes work.ExecCmd in the calculation of the cache.ActionID.
I tried removing the execCmd check in builderRunTest and everything worked as expected, cached results are cached returned if the -exec parameter matches. | NeedsDecision | low | Critical |
353,973,797 | godot | Script "PRELOAD" allocated memory are not freed until editor restart... and sometimes not even with that. | <!-- Please search existing issues for potential duplicates before filing yours:
https://github.com/godotengine/godot/issues?q=is%3Aissue
-->
Related issues at bottom....
**Godot version:**
<!-- Specify commit hash if non-official. -->
All
**OS/device including version:**
<!-- Specify GPU model and drivers if graphics-related. -->
All
**Issue description:**
<!-- What happened, and what was expected. -->
If you do some "preloads" of packed scenes in the script editor, memory is inmediately allocated. If you delete them, memory is still allocated, no mathers if you save the script after deleting the preloads, or if you close all scenes and scripts... memory is not freed until editor restart....
But real problem here is: If you don´t delete that preloads and maintain that in any script, in any part of the project, no mathers if the script is singleton or not, opened or not......, in every session the memory is allways allocated, and it shouldn´t.
**Steps to reproduce:**
Preload any packed scene in any script and look at windows-task administrator. Open godot, close godot, maintain preloads and close-open, close-open scripts with and without, put preloads in autoloads, in other scripts, open editor with all script closed.... etc... etc... etc... not project related. Always look at task manager and see what happend with godot memory...
Related :
https://github.com/godotengine/godot/issues/20529
https://github.com/godotengine/godot/issues/9815 | bug,topic:editor | low | Minor |
353,997,238 | pytorch | how can i run two model in a simple project? | I have two models trained with caffe2 - detectron , i have convert the detectron model( pkl) to caffe2 models(pbtxt), and i have test the models succsssfully.
but i need run the two models one by one in a same project , so i can get the infor of the image,
but here is the problem
how can i Realization the function ?
what i want realization most like this,
class get_info:
def _init_():
init the network
def classify_img():
get_img_info()
main():
model_a = get_info()
model_b = get_info()
for( listdir()):
model_a.classify_img()
model_b.classify_img()
but now i can't realization this , here is my code
class caffe2DetectronClassify:
def _create_input_blobs_for_net(self,net_def):
for op in net_def.op:
for blob_in in op.input:
if not workspace.HasBlob(blob_in):
workspace.CreateBlob(blob_in)
def __init__(self, path_model_init, path_model_weights, scale, max_size, mean_file,root_dir):
detectron_ops_lib = _get_detectron_ops_lib()
dyndep.InitOpsLibrary(detectron_ops_lib)
self.init_net = caffe2_pb2.NetDef()
with open(path_model_init, 'rb') as f:
self.init_net.ParseFromString(f.read())
self.net = caffe2_pb2.NetDef()
with open(path_model_weights, 'rb') as f:
self.net.ParseFromString(f.read())
self.scale = scale
self.max_size = max_size
self.mean_file = mean_file
**workspace.ResetWorkspace()**
workspace.RunNetOnce(self.init_net)
self._create_input_blobs_for_net(self.net)
workspace.CreateNet(self.net)
print("net create over ...")
def img_classify(self,img_path):
if(not os.path.exists(img_path)):
return [],[],[]
im = cv2.imread(img_path)
if(im.any() == None):
print('None image')
return [],[],[]
input_blob = self._prepare_blobs(im,self.mean_file,self.scale,self.max_size)
gpu_blobs = ['data']
for k,v in input_blob.items():
workspace.FeedBlob(
core.ScopedName(k),
v,
self._get_device_option_cuda() if k in gpu_blobs else
self._get_device_option_cpu()
)
try:
workspace.RunNet(self.net.name)
scores = workspace.FetchBlob('score_nms')
classids = workspace.FetchBlob('class_nms')
boxes = workspace.FetchBlob('bbox_nms')
except Exception as e:
print('error with {}'.format(e))
R = 0
scores = np.zeros((R,), dtype=np.float32)
boxes = np.zeros((R, 4), dtype=np.float32)
classids = np.zeros((R,), dtype=np.float32)
return scores,classids,boxes
when i run ,got error
Found Detectron ops lib: /home/jansonm/caffe2/pytorch/build/lib/libcaffe2_detectron_ops_gpu.so
WARNING: Logging before InitGoogleLogging() is written to STDERR
W0825 16:40:33.968032 21053 init.h:99] Caffe2 GlobalInit should be run before any other API calls.
W0825 16:40:34.370723 21053 init.h:99] Caffe2 GlobalInit should be run before any other API calls.
net create over ...
Found Detectron ops lib: /home/jansonm/caffe2/pytorch/build/lib/libcaffe2_detectron_ops_gpu.so
net create over ...
++++++++++++++++++++++++++++++++++++++++++++++++
/home/jansonm/detectron/test_img/5498233_1012130_1530585802475_10606738.jpeg
[]
pre imag time:1.59693694115
-----------------------------------------------
No handlers could be found for logger "caffe2.python.workspace"
error with [enforce fail at pybind_state.cc:1054] gWorkspace->GetNet(name). Can't find net detectron_detect_box
[]
pre imag time:0.141842126846
++++++++++++++++++++++++++++++++++++++++++++++++
sh: pause: command not found
++++++++++++++++++++++++++++++++++++++++++++++++
/home/jansonm/detectron/test_img/5498235_1023198_1530580487026_19862435.jpeg
[]
pre imag time:0.643126964569
-----------------------------------------------
error with [enforce fail at pybind_state.cc:1054] gWorkspace->GetNet(name). Can't find net detectron_detect_box
[]
pre imag time:0.144562005997
++++++++++++++++++++++++++++++++++++++++++++++++
sh: pause: command not found
you see , the second model can not work, can not found its model by name
i think it is because when init the model
**workspace.ResetWorkspace()**
workspace.RunNetOnce(self.init_net)
self._create_input_blobs_for_net(self.net)
workspace.CreateNet(self.net)
when i init workspace.ResetWorkspace() , it recover the first model, how can i let then work at the same time in a sample project,??
@eklitzke
@gchanan
| caffe2 | low | Critical |
354,004,352 | TypeScript | JSDoc @augments doesn’t allow function calls to augment existing types | I wrote a simple extension of [jest.Matchers][1] but I can not get the typescript type checker to recognize my extension.
<!-- Please try to reproduce the issue with `typescript@next`. It may have already been fixed. -->
**TypeScript Version:** 3.0.1 (and 3.1.0-dev.20180825)
The description below is for 3.0.1 - for 3.1.0-dev.20180825 all jest functions show errors, so I don't even think that the parser gets to my type at all.
```
[ts] Cannot find name 'describe'.
[ts] Cannot find name 'test'.
[ts] Cannot find name 'expect
```
Used via vscode version: 1.26.1
<!-- Search terms you tried before logging this (so others can find this issue more easily) -->
**Search Terms:**
is:issue is:open jsdoc extends
is:issue is:open jsdoc @augments
is:issue is:open jsdoc "@augments"
I first asked this as a [question on StackOverflow](https://stackoverflow.com/questions/51972275/jsdoc-document-object-method-extending-other-class) but after waiting a few days, I now suspect it is a bug in typescript's JSDoc implementation.
I also read the FAQ and found [the link](https://github.com/Microsoft/TypeScript/wiki/FAQ#how-do-i-write-unit-tests-with-typescript) to jest but didn't find any information there either.
I'm using plain JavaScript and the code runs perfectly.
**Code**
```js
// @ts-check
const getFunctorValue = F => {
let x
F.fmap(v => x = v)
return x
}
expect.extend({
/**
* @extends jest.Matchers
* @param {*} actual The functor you want to test.
* @param {*} expected The functor you expect.
*/
functorToBe(actual, expected) {
const actualValue = getFunctorValue(actual)
const expectedValue = getFunctorValue(expected)
const pass = Object.is(actualValue, expectedValue)
return {
pass,
message () {
return `expected ${actualValue} of ${actual} to ${pass ? '' : 'not'} be ${expectedValue} of ${expected}`
}
}
}
})
/**
* @param {*} v Any value
*/
function just (v) {
return {
fmap: f => just(f(v))
}
}
describe('Functor Law', () => {
test('equational reasoning (identity)', () => {
expect(just(1)).functorToBe(just(1))
})
})
```
**Expected behavior:**
`expect().functorToBe` should be recognized as a function.
**Actual behavior:**
But in the line with `expect(just(1)).functorToBe(just(1))`,
I get a red underline under `functorToBe` and the following error message:
>[ts] Property 'functorToBe' does not exist on type 'Matchers<{ [x: string]: any; fmap: (f: any) => any; }>'.
any
I got `jest.Matchers` from writing `expect()` in [vscode][2] and looked at the description.
[![image of vscode intellisense displaying jest.Matchers as return type for expect()][3]][3]
**Playground Link:** <!-- A link to a TypeScript Playground "Share" link which demonstrates this behavior -->
**Related Issues:** <!-- Did you find other bugs that looked similar? -->
No.
[1]: https://jestjs.io/docs/en/expect#expectextendmatchers
[2]: https://code.visualstudio.com/
[3]: https://i.stack.imgur.com/fvwKY.png | Suggestion,Domain: JSDoc,Domain: JavaScript,Experience Enhancement | low | Critical |
354,011,719 | godot | Node.set_display_folded(bool) has no effect | **Godot version:**
3.0.5-stable_x11.64, Xubuntu
**Issue description:**
_Node.set_display_folded(bool)_ has no effect whatsoever.
**Example project:**
[Example project.zip](https://github.com/godotengine/godot/files/2320724/Example.project.zip)
| bug,confirmed,topic:plugin | low | Major |
354,011,741 | vscode | Identation rules are broken | - VSCode Version: v1.26.1
- OS Version: macOS 10.13.5
I think I've had the following problems since the very first version of VSC I tried.
### Problem 1
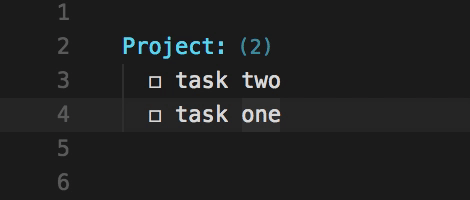
1. Have [Todo+](https://marketplace.visualstudio.com/items?itemName=fabiospampinato.vscode-todo-plus) installed and create a new "todo" document with the same content as mine.
2. Move the line up.
3. Undo.
4. Move the line up.
5. We get 2 different results for doing the same thing.
### Problem 2
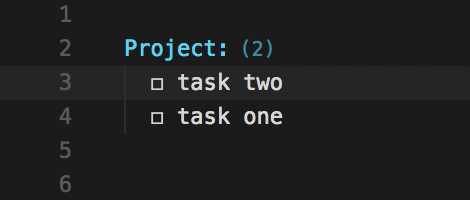
1. Have [Todo+](https://marketplace.visualstudio.com/items?itemName=fabiospampinato.vscode-todo-plus) installed and create a new "todo" document with the same content as mine.
2. Move the line down.
3. Undo.
4. Move the line down.
5. We get 2 different results for doing the same thing.
### Problem 3
In both the gifs above the indentation for a line gets decreased, my understanding is that this is governed by the [`indentationRules.decreaseIndentPattern`](https://github.com/fabiospampinato/vscode-todo-plus/blob/master/src/utils/init.ts/#L38-L38) setting, which in my extension is set to `/(?=a)b/gm`, a regex that can never match any string, why are those lines' indentations decreased then? | bug,verification-found,editor-autoindent | low | Critical |
354,017,067 | go | testing: tiny benchmark with StopTimer runs forever | From at least Go 1,4 onward,
```
$ go version
go version devel +e03220a594 Sat Aug 25 02:39:49 2018 +0000 linux/amd64
$
```
If we run a tiny benchmark (count++) and use StopTimer() for initialization (count = 0) then the benchmark runs for a very long time, perhaps forever.
```
$ cat tiny_test.go
package main
import (
"testing"
)
var count int64
func BenchmarkTiny(b *testing.B) {
for n := 0; n < b.N; n++ {
b.StopTimer()
count = 0
b.StartTimer()
count++
}
}
$
```
```
$ go test tiny_test.go -bench=.
goos: linux
goarch: amd64
BenchmarkTiny-4 ^Csignal: interrupt
FAIL command-line-arguments 143.633s
$
```
If we comment out StopTimer() (//b.StopTimer()) then the benchmark quickly runs to completion.
```
$ cat tiny_test.go
package main
import (
"testing"
)
var count int64
func BenchmarkTiny(b *testing.B) {
for n := 0; n < b.N; n++ {
// b.StopTimer()
count = 0
b.StartTimer()
count++
}
}
$
```
```
$ go test tiny_test.go -bench=.
goos: linux
goarch: amd64
BenchmarkTiny-4 1000000000 2.15 ns/op
PASS
ok command-line-arguments 2.374s
$
```
| NeedsInvestigation | low | Major |
354,018,187 | go | doc: cmd/go: some module docs are on swtch.com, not golang.org | Please answer these questions before submitting your issue. Thanks!
### What version of Go are you using (`go version`)?
golang.org today
### Does this issue reproduce with the latest release?
yes
### What operating system and processor architecture are you using (`go env`)?
I don't think it matters
### What did you do?
went to golang.org
If possible, provide a recipe for reproducing the error.
A complete runnable program is good.
A link on play.golang.org is best.
### What did you expect to see?
Documentation for the go programming language to be hosted on golang.org
### What did you see instead?
Documentation for go modules hosted on swtch.com
| Documentation,NeedsFix,modules | low | Critical |
354,040,047 | rust | Captureless closures can still codegen to `fn call(&self)` / `fn call_mut(&mut self)`. | Despite them being ZSTs and `Copy`, which would allow `Fn` / `FnMut` impls on top of `FnOnce`.
This small example:
```rust
fn foo(f: impl FnOnce()) { f() }
pub fn test() {
// Not passed directly in call to avoid inferring `closure` to `FnOnce`.
let closure = || {};
foo(closure);
}
```
will cause a `ClosureOnceShim` to be generated, which takes the ZST closure, borrows it, and passes it to the actual closure "function", that has been inferred to be `Fn` (I think?).
While correct, it's an extra function between the caller and the closure, and there's a potential risk of LLVM doing not-that-nice (e.g. inlining-related) things (and mergefunc might not work here).
This seems tricky to fix, since parts of the compiler *assume* that e.g. a closure implements `FnMut` if it can be called with a mutable reference to its environment, and if it implements `FnMut` *and not* `Fn`, then its "codegen'd function" *must* have a `FnMut::call_mut` signature.
cc @rust-lang/wg-codegen | C-enhancement,A-codegen,T-compiler,WG-llvm | low | Minor |
354,067,926 | flutter | Add arrows to assets-for-api-docs utils | c: new feature,team,framework,d: api docs,a: assets,P3,team-framework,triaged-framework | low | Minor |
|
354,068,013 | flutter | Make it easier to run diagrams in assets-for-api-docs with hot reload | Could be just adding a blank flutter project that makes it easy to runApp(<diagram>) | a: tests,team,framework,d: api docs,P2,team-framework,triaged-framework | low | Minor |
354,079,593 | vscode | Feature Request: Hotkey Support for QuickInputButton | <!-- Please search existing issues to avoid creating duplicates. -->
Issue Type: **Feature Request**
<!-- Describe the feature you'd like. -->
As an extension developer I want to assign a hotkey to a custom [QuickInputButton](https://code.visualstudio.com/docs/extensionAPI/vscode-api#QuickInputButton), that is active/enabled when the [InputBox](https://code.visualstudio.com/docs/extensionAPI/vscode-api#InputBox) or [QuickPick<T>](https://code.visualstudio.com/docs/extensionAPI/vscode-api#QuickPick%3CT%3E) is shown. This would enhance navigating through multi step input forms a lot. It should have similar behaviour like the [QuickInputButtons.Back](https://code.visualstudio.com/docs/extensionAPI/vscode-api#QuickInputButtons) button which is by default assigned to <kbd>Alt</kbd> + <kbd>←</kbd>. Further I want to allow the extension user to assign his/her preferred hotkey to that button, too. Therefore maybe "some link" to the `keybindings.json` would be applicable. | feature-request,quick-pick | low | Major |
354,080,759 | rust | Option as_ref compilation error when implementing cause method for Error trait | Hi, I'm new to rust and this looks like a possible bug to me. I implemented my own error type (focus on the cause method implementation in Error trait):
```
#[derive(Debug)]
pub struct MyError<T: Error>
{
description: String,
cause: Option<T>
}
impl <T: Error> fmt::Display for MyError<T> {
fn fmt(&self, f: &mut fmt::Formatter) -> fmt::Result {
write!(f, "{}", self.description)
}
}
impl <T: Error> MyError<T> {
pub fn new(description: String, cause: Option<T>) -> MyError<T> {
MyError{description, cause}
}
}
impl <T: Error> Error for MyError<T> {
fn description(&self) -> &str {
self.description.as_str()
}
fn cause(&self) -> Option<&Error> {
// doesn't work
//self.cause.as_ref()
//does work
match self.cause {
None => None,
Some(ref e)=> Some(e)
}
}
}
```
This code seems to work correctly but when I use the commented `self.cause.as_ref()` instead of the match clause I'm getting following compilation error:
```
error[E0308]: mismatched types
--> src\lib.rs:72:9
|
72 | self.cause.as_ref()
| ^^^^^^^^^^^^^^^^^^^ expected trait std::error::Error, found type parameter
|
= note: expected type `std::option::Option<&std::error::Error>`
found type `std::option::Option<&T>`
```
I would normally expect it to work because the working match I use as workaround is pretty much the same as the underlying implementation of [as_ref() in Option](https://github.com/rust-lang/rust/blob/master/src/libcore/option.rs#L245).
## Meta
λ rustc --version --verbose
rustc 1.28.0 (9634041f0 2018-07-30)
binary: rustc
commit-hash: 9634041f0e8c0f3191d2867311276f19d0a42564
commit-date: 2018-07-30
host: x86_64-pc-windows-msvc
release: 1.28.0
LLVM version: 6.0 | A-trait-system,T-compiler | low | Critical |
354,090,590 | godot | Default Contextual Help keyboard shortcut doesn't work due to conflicts with macOS keyboard handling | **Godot version:**
3.0.6
**OS/device including version:**
macOS High Sierra 10.13.6
**Issue description:**
The keyboard shortcut `Shift+Alt+Space` doesn't display contextual help. Instead the editor inserts a non-breaking space as if `Alt+Space` was pressed without the `Shift`
Contextual Help works when selected from the menu.
**Steps to reproduce:**
1. open script editor
2. press `Shift+Alt+Space`
**Minimal reproduction project:**
N/A
| bug,platform:macos,topic:editor,confirmed | low | Major |
354,090,748 | go | cmd/compile: mipsle: running error SIGILL: illegal instruction | Please answer these questions before submitting your issue. Thanks!
### What version of Go are you using (`go version`)?
go version go1.11 linux/amd64
### Does this issue reproduce with the latest release?
yes
### What operating system and processor architecture are you using (`go env`)?
build on : amd64 linux,
runing on: Linux OpenWrt 4.4.14 #1 Mon May 29 15:04:41 UTC 2017 mips GNU/Linux
### What did you do?
file from:
https://github.com/eclipse/paho.mqtt.golang/tree/master/cmd/simple/main.go
build command:
`env GOOS=linux GOARCH=mipsle GOMIPS=softfloat go build -ldflags "-s -w" `
runing on openwrt system,32 bit os. router.
MT7620A 16M ROM, 128M RAM
```
root@OpenWrt:~# ./simple
SIGILL: illegal instruction
PC=0x13ebac m=0 sigcode=128
goroutine 1 [running, locked to thread]:
math/big.init()
/usr/local/go/src/math/big/sqrt.go:10 +0xbc fp=0xc22780 sp=0xc22770 pc=0x13ebac
crypto/elliptic.init()
<autogenerated>:1 +0x88 fp=0xc22784 sp=0xc22780 pc=0x14b150
crypto/ecdsa.init()
<autogenerated>:1 +0x98 fp=0xc22798 sp=0xc22784 pc=0x15bd60
crypto/tls.init()
<autogenerated>:1 +0x90 fp=0xc227b4 sp=0xc22798 pc=0x1b0568
github.com/eclipse/paho%2emqtt%2egolang.init()
<autogenerated>:1 +0xc0 fp=0xc227c8 sp=0xc227b4 pc=0x237eb4
main.init()
<autogenerated>:1 +0xa0 fp=0xc227cc sp=0xc227c8 pc=0x239400
runtime.main()
/usr/local/go/src/runtime/proc.go:189 +0x1f8 fp=0xc227ec sp=0xc227cc pc=0x431dc
runtime.goexit()
/usr/local/go/src/runtime/asm_mipsx.s:660 +0x4 fp=0xc227ec sp=0xc227ec pc=0x76b70
r0 0x0 r1 0x2
r2 0x1 r3 0x1
r4 0x8ece6c r5 0x1
r6 0x4 r7 0x54
r8 0x27a0e0 r9 0x2f2e46
r10 0x8 r11 0x0
r12 0x51 r13 0x1f
r14 0x1 r15 0x52
r16 0x0 r17 0x80000000
r18 0xff r19 0x6fa46804
r20 0xc0c288 r21 0xc0c288
r22 0x2c1508 r23 0x2f0000
r24 0x2 r25 0x0
r26 0x0 r27 0x0
r28 0xfffff000 r29 0xc22770
r30 0xc000e0 r31 0x13eba8
pc 0x13ebac link 0x13eba8
lo 0x0 hi 0x0
```
| NeedsInvestigation | low | Critical |
354,097,200 | flutter | ModalRoute / ModalBarrier customization | Currently, `ModalRoute`s created using `showDialog` or `showGeneralDialog` do not allow full customization of the underlying `ModalBarrier`.
It’s only possible to change the background color, however I’d like full control over the animated widget in order to build my own. For example, a customer requested to apply Gaussian blur to the barrier.
The current solution involves creating the barrier myself and not using the neat routing system.
So the same way that `showGeneralRoute` has a `PageBuilder` Parameter, it could gain a `BarrierBuilder`. | c: new feature,framework,f: routes,P2,team-framework,triaged-framework | low | Major |
354,106,195 | rust | Two-phase borrows don't allow mutably splitting an array based on its length | ```rust
#![feature(nll)]
fn main() {
let mut x = [1, 2];
x.split_at_mut(x.len() / 2);
}
```
```
error[E0502]: cannot borrow `x` as immutable because it is also borrowed as mutable
--> src/main.rs:4:20
|
4 | x.split_at_mut(x.len() / 2);
| ---------------^-----------
| | |
| | immutable borrow occurs here
| mutable borrow occurs here
| borrow later used here
```
---
1.30.0-nightly (2018-08-24 d41f21f11a249ad78990)
/cc @nikomatsakis @pnkfelix | P-medium,T-compiler,A-NLL,NLL-complete,A-array | low | Critical |
354,123,549 | rust | `pub use serde::*` doesn't show traits in `cargo doc` | This is somewhat weird, but on https://konradborowski.gitlab.io/serde-feature-hack/serde_feature_hack/index.html, I don't see traits like `Serialize`, even if they should be here, and there is no note of re-export either. On https://docs.rs/serde-feature-hack/0.1.0/serde_feature_hack/, it shows a re-export, so this appears to be a regression.
The code:
```rust
extern crate serde;
pub use serde::*;
``` | T-rustdoc,E-needs-test,C-bug | low | Minor |
354,132,892 | godot | Built-in Array types don't open in a new inspector if their name is added to the "Resource Types To Open In New Inspector" editor setting | **Godot version:**
v3.1.dev.calinou.5df9109
**OS/device including version:**
Windows 10 Home v1803
**Issue description:**
Any of the built-in array types (Array or Pool\*Array) do not open in a new inspector, even if the "Open Resources In New Inspector" option is checked, and even if the array types are added to "Resource Types To Open In New Inspector".
Not being able to open them in a new inspector window prevents me from using "Copy Params" and "Paste Params" on the Array types. This means that, if for example I need to copy over a array of integers, I currently have to do it by hand. That can get tedious quick.
**Steps to reproduce:**
1. Set the inspector to always use a new inspector window.
2. Create a script that exports an Array or any of the Pool\*Array types.
3. Click on them in the inspector and notice that they don't open in a new inspector window.
**Minimal reproduction project:**
Should be pretty easy to check, just chuck any of the lines from this into a script:
```gdscript
export(Array) var array
export(PoolByteArray) var bytes
export(PoolColorArray) var colors
export(PoolIntArray) var ints
export(PoolRealArray) var reals
export(PoolStringArray) var strings
export(PoolVector2Array) var vector2s
export(PoolVector3Array) var vector3s
```
And then try to open them in a new inspector window. | bug,topic:editor | low | Major |
354,175,733 | rust | Rustc hangs during llvm codegen when referencing a [large] array with std::ptr::read_volatile | This issue has been repro'd on stable and nightly on Windows, OSX, and Linux:
```
cargo 1.28.0 (96a2c7d16 2018-07-13)
release: 1.28.0
commit-hash: 96a2c7d16249cb47c61c887fc95ca8be60e7ef0a
commit-date: 2018-07-13
cargo 1.29.0-nightly (6a7672ef5 2018-08-14)
release: 1.29.0
commit-hash: 6a7672ef5344c1bb570610f2574250fbee932355
commit-date: 2018-08-14
```
Code:
```
fn main() {
let x = [0u8; 128 * 128 * 4];
let _y = unsafe { std::ptr::read_volatile(&x) };
}
```
Thanks to @talchas for the minimal repro. I believe they were able to repro it without `_volatile` as well. | A-LLVM,T-compiler | low | Minor |
354,234,031 | opencv | Feature request: Allow a PR contributor to restart specific-platform build | In case of unexpected platform failure, we usually perform `commit --amend` to restart the tests, but this affects all the platforms.
Cannot you provide a way, say a button inside each cell of a row belonging to owned PRs, to restart specific builds? I guess the admins has such facility, but why not to provide that to the contributors as well?
BTW: Is there any guide for the whole list of build platforms specific-names (e.g. `ocllinux`, `win32`) that we can utilize inside `force_builders`? For example, how to turn `Win64 MSVS2017` on? | category: infrastructure | low | Critical |
354,238,988 | TypeScript | Add API to query if SourceFile originated from typeRoots lookup | This proposes to add an API `Program#isSourceFileFromTypeRoots` that is similar to `Program#isSourceFileFromExternalLibrary` but for `typeRoots`.
Currently the only way to determine if a SourceFile comes from `typeRoots` is to use `ts.getEffectiveTypeRoots` and check if a given `SourceFile#fileName` starts with any of the paths.
That works if there are no symlinks. With symlinks one needs to use `realpath` on all of the `typeRoots` before comparing to `fileName` as that is already a realpath.
And even that doesn't cover cases where a subdirectory (like `node_modules/@types/foo`) or an individual file (`node_modules/@types/foo/index.d.ts`) is a symlink.
Related: https://github.com/fimbullinter/wotan/issues/387 | Suggestion,In Discussion,API | low | Minor |
354,283,277 | tensorflow | Search in Embedding Projector using Japanese or Hindi text causes Cannot read property 'toString' of undefined | Please go to Stack Overflow for help and support:
https://stackoverflow.com/questions/tagged/tensorflow
If you open a GitHub issue, here is our policy:
1. It must be a bug, a feature request, or a significant problem with documentation (for small docs fixes please send a PR instead).
2. The form below must be filled out.
3. It shouldn't be a TensorBoard issue. Those go [here](https://github.com/tensorflow/tensorboard/issues).
**Here's why we have that policy**: TensorFlow developers respond to issues. We want to focus on work that benefits the whole community, e.g., fixing bugs and adding features. Support only helps individuals. GitHub also notifies thousands of people when issues are filed. We want them to see you communicating an interesting problem, rather than being redirected to Stack Overflow.
------------------------
### System information
Visiting http://projector.tensorflow.org/?config=https://ecraft2learn.github.io/ai/word-embeddings/hi/projector.json using Chrome 68
- **Have I written custom code (as opposed to using a stock example script provided in TensorFlow)**:
- **OS Platform and Distribution (e.g., Linux Ubuntu 16.04)**:
- **Mobile device (e.g. iPhone 8, Pixel 2, Samsung Galaxy) if the issue happens on mobile device**:
- **TensorFlow installed from (source or binary)**:
- **TensorFlow version (use command below)**:
- **Python version**:
- **Bazel version (if compiling from source)**:
- **GCC/Compiler version (if compiling from source)**:
- **CUDA/cuDNN version**:
- **GPU model and memory**:
- **Exact command to reproduce**:
You can collect some of this information using our environment capture script:
https://github.com/tensorflow/tensorflow/tree/master/tools/tf_env_collect.sh
You can obtain the TensorFlow version with
python -c "import tensorflow as tf; print(tf.GIT_VERSION, tf.VERSION)"
### Describe the problem
After visiting http://projector.tensorflow.org/?config=https://ecraft2learn.github.io/ai/word-embeddings/hi/projector.json using Chrome 68 and then entering any Hindi text in the Search field the console shows
Uncaught TypeError: Cannot read property 'toString' of undefined
at b (?config=https://ecraft2learn.github.io/ai/word-embeddings/hi/projector.json:formatted:60928)
at ?config=https://ecraft2learn.github.io/ai/word-embeddings/hi/projector.json:formatted:61345
at Array.forEach (<anonymous>)
at a.query (?config=https://ecraft2learn.github.io/ai/word-embeddings/hi/projector.json:formatted:61344)
at ?config=https://ecraft2learn.github.io/ai/word-embeddings/hi/projector.json:formatted:66646
at ?config=https://ecraft2learn.github.io/ai/word-embeddings/hi/projector.json:formatted:66171
at Array.forEach (<anonymous>)
at HTMLElement.b.notifyInputChanged (?config=https://ecraft2learn.github.io/ai/word-embeddings/hi/projector.json:formatted:66170)
at HTMLElement.b.onTextChanged (?config=https://ecraft2learn.github.io/ai/word-embeddings/hi/projector.json:formatted:66185)
at HTMLElement.<anonymous> (?config=https://ecraft2learn.github.io/ai/word-embeddings/hi/projector.json:formatted:66151)
b @ ?config=https://ecraft2learn.github.io/ai/word-embeddings/hi/projector.json:formatted:60928
(anonymous) @ ?config=https://ecraft2learn.github.io/ai/word-embeddings/hi/projector.json:formatted:61345
a.query @ ?config=https://ecraft2learn.github.io/ai/word-embeddings/hi/projector.json:formatted:61344
(anonymous) @ ?config=https://ecraft2learn.github.io/ai/word-embeddings/hi/projector.json:formatted:66646
(anonymous) @ ?config=https://ecraft2learn.github.io/ai/word-embeddings/hi/projector.json:formatted:66171
b.notifyInputChanged @ ?config=https://ecraft2learn.github.io/ai/word-embeddings/hi/projector.json:formatted:66170
b.onTextChanged @ ?config=https://ecraft2learn.github.io/ai/word-embeddings/hi/projector.json:formatted:66185
(anonymous) @ ?config=https://ecraft2learn.github.io/ai/word-embeddings/hi/projector.json:formatted:66151
### Source code / logs
Include any logs or source code that would be helpful to diagnose the problem. If including tracebacks, please include the full traceback. Large logs and files should be attached. Try to provide a reproducible test case that is the bare minimum necessary to generate the problem.
| comp:tensorboard,stat:awaiting tensorflower,type:feature | low | Critical |
354,295,514 | rust | Experiment with a "(type) pattern pre-compiler" in rustc::ty::_match. | Instead of zipping two trees and using the same unification procedure as the rest of inference, the "pattern" type could be turned into linear "instruction stream" for a matcher ("interpreter"), to walk the type "tree", first to check that the type actually matches and *then* to unify variables (if any).
E.g for. `Result<Vec<$0>, io::Error>`, it would check the `Result`, `Vec` and `io::Error` heads, and for `($0, $0)` it could equate the pair elements, *before* unifying a type with `$0`, in both cases.
This could also apply to Chalk, but I'm less familiar with its internal representation.
cc @nikomatsakis @sunjay | C-enhancement,T-compiler,A-inference | low | Critical |
354,351,548 | pytorch | LNK2019 error when linking with MSVC [Caffe2] | ## Issue description
I've been trying to build a simple C++ program using the Caffe2 library (statically linked) since last week and it has been a painful experience. I couldn't find any information on how to properly build the Caffe2 library so I've had to figure it out through trial and error. I managed to compile and install the caffe2 lib with these commands (powershell):
```
> cd pytorch
> mkdir build
> cd build
> cmake ../.. -DCMAKE_INSTALL_PREFIX="${HOME}/caffe2-static-minimal" -DBUILD_PYTHON=OFF -DUSE_CUDA=OFF -DUSE_GFLAGS=OFF -DUSE_GLOG=OFF -DUSE_GLOO=OFF -DBUILD_SHARED_LIBS=OFF -DBUILD_TEST=OFF -DBUILD_BINARY=OFF -DUSE_LMDB=OFF -DUSE_LEVELDB=OFF -DUSE_MPI=OFF -DUSE_OPENMP=OFF -DUSE_OPENCV=OFF -DBUILD_ATEN=ON -DCAFFE2_DISABLE_NUMA=1 -G "Visual Studio 15 2017 Win64"
> cmake --build . --config Release --target install
> # For some reason the caffe2_protos.lib and onnxifi_loader.lib files aren't installed, so I've to manually copy then:
> cp lib/Release/caffe2_protos.lib "${HOME}/caffe2-static-minimal/lib/"
> cp lib/Release/onnxifi_loader.lib "${HOME}/caffe2-static-minimal/lib/"
> # The protobuf library shouldn't start with 'lib' when building in a Windows enviroment:
> pushd "${HOME}/caffe2-static-minimal/lib/"
> mv libprotobuf.lib protobuf.lib
> popd
```
When I try to build the simple example listed bellow I get a bunch of link errors, MSVC output:
```
(Link target) ->
test-caffe2.obj : error LNK2019: unresolved external symbol "__declspec(dllimport) private: __cdecl caffe2::TypeIdentifier::TypeIdentifier(unsigned short)" (__imp_??0TypeIdentifier@caffe2@@AEAA@G@Z) referenced in function "public: __cdecl caffe2::Tensor::Tensor<float>(class std::vector<__int64,class std::allocator<__int64> > const &,class std::vector<float,class std::allocator<float> > const &,class caffe2::BaseContext *)" (??$?0M@Tensor@caffe2@@QEAA@AEBV?$vector@_JV?$allocator@_J@std@@@std@@AEBV?$vector@MV?$allocator@M@std@@@3@PEAVBaseContext@1@@Z) [C:\Users\Administrator\repos\test-caffe2\build\test-caffe2.vcxproj]
test-caffe2.obj : error LNK2019: unresolved external symbol "__declspec(dllimport) private: __cdecl caffe2::TypeMeta::TypeMeta(class caffe2::TypeIdentifier,unsigned __int64,void (__cdecl*)(void *,unsigned __int64),void (__cdecl*)(void const *,void *,unsigned __int64),void (__cdecl*)(void *,unsigned __int64))" (__imp_??0TypeMeta@caffe2@@AEAA@VTypeIdentifier@1@_KP6AXPEAX1@ZP6AXPEBX21@Z3@Z) referenced in function "public: __cdecl caffe2::Tensor::Tensor<float>(class std::vector<__int64,class std::allocator<__int64> > const &,class std::vector<float,class std::allocator<float> > const &,class caffe2::BaseContext *)" (??$?0M@Tensor@caffe2@@QEAA@AEBV?$vector@_JV?$allocator@_J@std@@@std@@AEBV?$vector@MV?$allocator@M@std@@@3@PEAVBaseContext@1@@Z) [C:\Users\Administrator\repos\test-caffe2\build\test-caffe2.vcxproj]
test-caffe2.obj : error LNK2019: unresolved external symbol "__declspec(dllimport) public: unsigned __int64 const & __cdecl caffe2::TypeMeta::itemsize(void)const " (__imp_?itemsize@TypeMeta@caffe2@@QEBAAEB_KXZ) referenced in function "public: __cdecl caffe2::Tensor::Tensor<float>(class std::vector<__int64,class std::allocator<__int64> > const &,class std::vector<float,class std::allocator<float> > const &,class caffe2::BaseContext *)" (??$?0M@Tensor@caffe2@@QEAA@AEBV?$vector@_JV?$allocator@_J@std@@@std@@AEBV?$vector@MV?$allocator@M@std@@@3@PEAVBaseContext@1@@Z) [C:\Users\Administrator\repos\test-caffe2\build\test-caffe2.vcxproj]
test-caffe2.obj : error LNK2019: unresolved external symbol "__declspec(dllimport) public: void (__cdecl*__cdecl caffe2::TypeMeta::ctor(void)const )(void *,unsigned __int64)" (__imp_?ctor@TypeMeta@caffe2@@QEBAP6AXPEAX_K@ZXZ) referenced in function "public: void * __cdecl caffe2::Tensor::raw_mutable_data(class caffe2::TypeMeta const &)" (?raw_mutable_data@Tensor@caffe2@@QEAAPEAXAEBVTypeMeta@2@@Z) [C:\Users\Administrator\repos\test-caffe2\build\test-caffe2.vcxproj]
test-caffe2.obj : error LNK2019: unresolved external symbol "__declspec(dllimport) public: void (__cdecl*__cdecl caffe2::TypeMeta::copy(void)const )(void const *,void *,unsigned __int64)" (__imp_?copy@TypeMeta@caffe2@@QEBAP6AXPEBXPEAX_K@ZXZ) referenced in function "public: __cdecl caffe2::Tensor::Tensor<float>(class std::vector<__int64,class std::allocator<__int64> > const &,class std::vector<float,class std::allocator<float> > const &,class caffe2::BaseContext *)" (??$?0M@Tensor@caffe2@@QEAA@AEBV?$vector@_JV?$allocator@_J@std@@@std@@AEBV?$vector@MV?$allocator@M@std@@@3@PEAVBaseContext@1@@Z) [C:\Users\Administrator\repos\test-caffe2\build\test-caffe2.vcxproj]
test-caffe2.obj : error LNK2019: unresolved external symbol "__declspec(dllimport) public: void (__cdecl*__cdecl caffe2::TypeMeta::dtor(void)const )(void *,unsigned __int64)" (__imp_?dtor@TypeMeta@caffe2@@QEBAP6AXPEAX_K@ZXZ) referenced in function "public: void * __cdecl caffe2::Tensor::raw_mutable_data(class caffe2::TypeMeta const &)" (?raw_mutable_data@Tensor@caffe2@@QEAAPEAXAEBVTypeMeta@2@@Z) [C:\Users\Administrator\repos\test-caffe2\build\test-caffe2.vcxproj]
C:\Users\Administrator\repos\test-caffe2\build\Release\test-caffe2.exe : fatal error LNK1120: 6 unresolved externals [C:\Users\Administrator\repos\test-caffe2\build\test-caffe2.vcxproj]
45 Warning(s)
7 Error(s)
```
I've tried applying [#10652](https://github.com/pytorch/pytorch/pull/10652) and [#10765](https://github.com/pytorch/pytorch/pull/10765) but I've got the same results.
## Code example
Here is a minimal example to reproduce the link problems:
test-caffe2.cpp:
```
#include <caffe2/core/init.h>
#include <caffe2/core/net.h>
#include <caffe2/utils/proto_utils.h>
int main(int argc, char **argv) {
caffe2::GlobalInit();
std::vector<float> data;
std::vector<caffe2::TIndex> dims({1, 3, 224, 224});
caffe2::CPUContext context;
volatile caffe2::Tensor tensor(dims, data, &context);
google::protobuf::ShutdownProtobufLibrary();
return 0;
}
```
CMakeLists.txt:
```
cmake_minimum_required(VERSION 2.6)
project (caffe2_test)
set(CMAKE_CXX_FLAGS_RELEASE "${CMAKE_CXX_FLAGS_RELEASE} /MT")
find_package(Threads)
set(ALL_LIBRARIES)
list(APPEND ALL_LIBRARIES ${CMAKE_THREAD_LIBS_INIT})
find_library(CAFFE2_LIB caffe2)
list(APPEND ALL_LIBRARIES ${CAFFE2_LIB})
find_library(PROTOBUF_LIB protobuf)
list(APPEND ALL_LIBRARIES ${PROTOBUF_LIB})
find_library(ONNX_PROTO_LIB onnx_proto)
list(APPEND ALL_LIBRARIES ${ONNX_PROTO_LIB})
find_library(ONNX_LIB onnx)
list(APPEND ALL_LIBRARIES ${ONNX_LIB})
find_library(ONNXIFI_LOADER_LIB onnxifi_loader)
list(APPEND ALL_LIBRARIES ${ONNXIFI_LOADER_LIB})
find_library(NOMNIGRAPH_LIB nomnigraph)
list(APPEND ALL_LIBRARIES ${NOMNIGRAPH_LIB})
find_library(CAFFE2_PROTO_LIB caffe2_protos)
list(APPEND ALL_LIBRARIES ${CAFFE2_PROTO_LIB})
find_library(CPUINFO_LIB cpuinfo)
list(APPEND ALL_LIBRARIES ${CPUINFO_LIB})
find_library(CLOG_LIB clog)
list(APPEND ALL_LIBRARIES ${CLOG_LIB})
include_directories(${CAFFE2_INCLUDE_DIR})
if(NOT CAFFE2_LIB)
message(FATAL_ERROR "Caffe2 lib not found")
endif()
set(CMAKE_CXX_STANDARD 11)
add_executable(test-caffe2 test-caffe2.cpp)
target_link_libraries(test-caffe2 ${ALL_LIBRARIES} ${CMAKE_DL_LIBS})
```
Build instructions (powershell):
```
> mkdir build
> cd build
> cmake .. -DCMAKE_PREFIX_PATH="${HOME}/caffe2-static-minimal/lib" -DCAFFE2_INCLUDE_DIR="${HOME}/caffe2-static-minimal/include/" -DCMAKE_BUILD_TYPE=Release -G "Visual Studio 15 2017 Win64"
> cmake --build . --config Release
```
Any hints?
## System Info
- PyTorch / Caffe2: c8b246abf31a8717105622077bc7669e2dc753a9 with [#10652](https://github.com/pytorch/pytorch/pull/10652) and [#10765](https://github.com/pytorch/pytorch/pull/10765) applyed
- Build command: ```cmake ../.. -DCMAKE_INSTALL_PREFIX="${HOME}/caffe2-static-minimal" -DBUILD_PYTHON=OFF -DUSE_CUDA=OFF -DUSE_GFLAGS=OFF -DUSE_GLOG=OFF -DUSE_GLOO=OFF -DBUILD_SHARED_LIBS=OFF -DBUILD_TEST=OFF -DBUILD_BINARY=OFF -DUSE_LMDB=OFF -DUSE_LEVELDB=OFF -DUSE_MPI=OFF -DUSE_OPENMP=OFF -DUSE_OPENCV=OFF -DBUILD_ATEN=ON -DCAFFE2_DISABLE_NUMA=1 -G "Visual Studio 15 2017 Win64"```
- OS: Windows Server 2016 1607
- MSVC version: 19.15.26726.0
- CMake version: 3.11.18040201-MSVC_2
| caffe2 | low | Critical |
354,400,455 | puppeteer | Print Screen Dialog is not caught by page.on("dialog") | 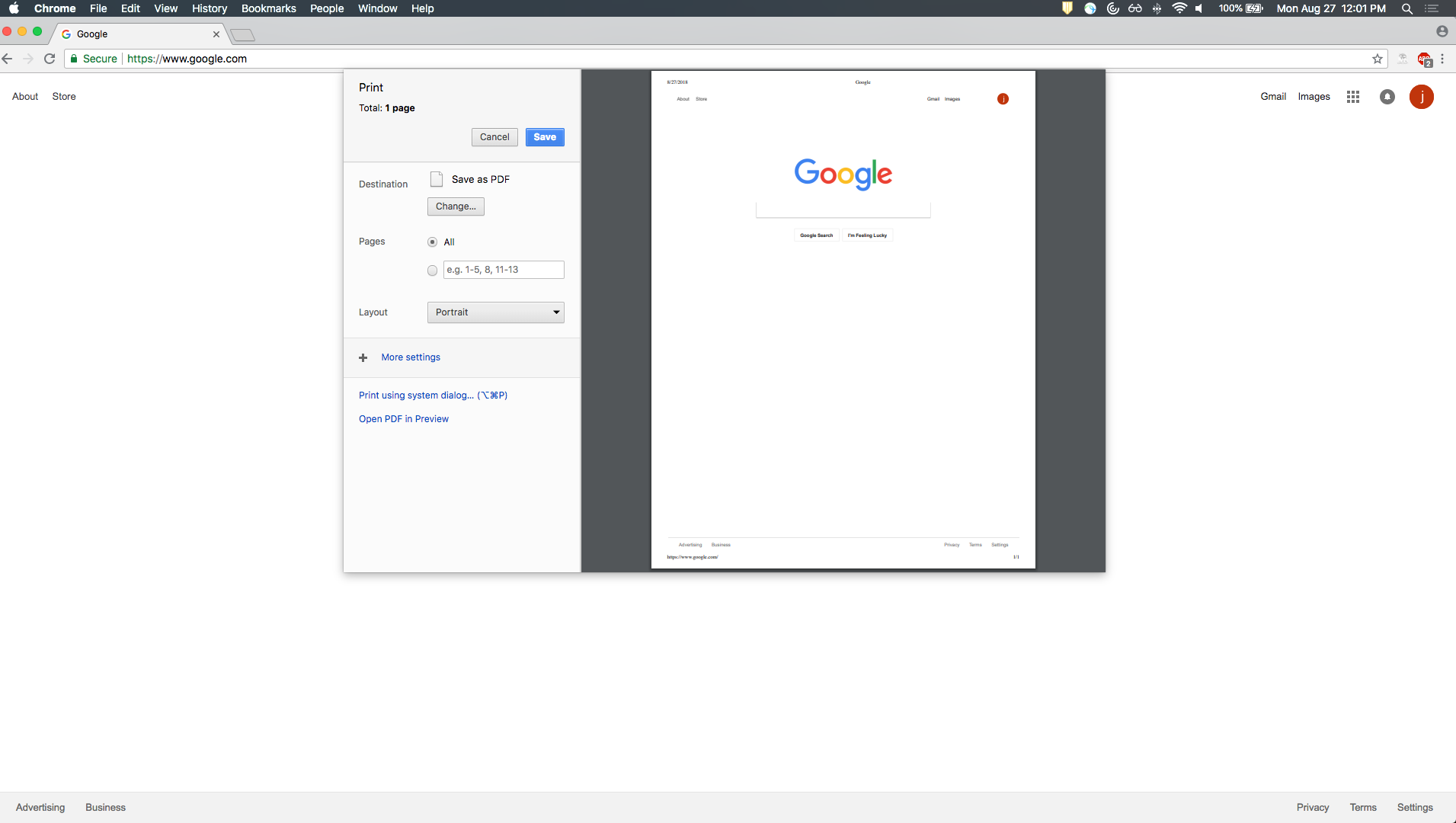
**Expected Result**
The console should log the dialog's message and type (in this case: the "print screen" dialog). I wanted to programmatically dismiss the print screen dialog.
**What happens instead?**
Nothing happens
**Environment:**
Puppeteer version: 1.7.0
Platform / OS version: Mac OSX Version 10.13.6
Node.js version: 10.7.0
**Code**
page = await browser.newPage();
page.on("dialog", async dialog => {
console.log("the dialog message is", dialog.message());
console.log("the dialog type is", dialog.type());
await dialog.dismiss();
});
await page.waitFor('.print-text'); //this clicks an element and opens up the print screen in google chrome
await page.click('.print-text');
| feature,upstream,chromium | medium | Critical |
354,402,715 | go | x/image/font: rendering texts in Arabic | ### What version of Go are you using (`go version`)?
```
go version go1.11 darwin/amd64
```
### Does this issue reproduce with the latest release?
Yes
### What operating system and processor architecture are you using (`go env`)?
```
GOARCH="amd64"
GOBIN=""
GOCACHE="/Users/hajimehoshi/Library/Caches/go-build"
GOEXE=""
GOFLAGS=""
GOHOSTARCH="amd64"
GOHOSTOS="darwin"
GOOS="darwin"
GOPATH="/Users/hajimehoshi/go"
GOPROXY=""
GORACE=""
GOROOT="/usr/local/go"
GOTMPDIR=""
GOTOOLDIR="/usr/local/go/pkg/tool/darwin_amd64"
GCCGO="gccgo"
CC="clang"
CXX="clang++"
CGO_ENABLED="1"
GOMOD="/Users/hajimehoshi/fonttest/go.mod"
CGO_CFLAGS="-g -O2"
CGO_CPPFLAGS=""
CGO_CXXFLAGS="-g -O2"
CGO_FFLAGS="-g -O2"
CGO_LDFLAGS="-g -O2"
PKG_CONFIG="pkg-config"
GOGCCFLAGS="-fPIC -m64 -pthread -fno-caret-diagnostics -Qunused-arguments -fmessage-length=0 -fdebug-prefix-map=/var/folders/b7/w11sqqrx7kx6fqfbn24wdsmh0000gn/T/go-build758210799=/tmp/go-build -gno-record-gcc-switches -fno-common"
```
### What did you do?
I tested to render a text in Arabic language:
```go
package main
import (
"flag"
"image"
"image/color"
"image/draw"
"image/png"
"io/ioutil"
"os"
"strings"
"github.com/golang/freetype/truetype"
"github.com/pkg/browser"
"golang.org/x/image/font"
"golang.org/x/image/math/fixed"
)
const (
// https://www.unicode.org/udhr/
text = "قوقحلاو ةماركلا يف نيواستم ارًارحأ سانلا عيمج دلوي."
)
func run() error {
const (
ox = 16
oy = 16
)
width := 640
height := 16*len(strings.Split(strings.TrimSpace(text), "\n")) + 8
dst := image.NewRGBA(image.Rect(0, 0, width, height))
draw.Draw(dst, dst.Bounds(), image.NewUniform(color.White), image.ZP, draw.Src)
// I tested an arg "/Library/Fonts/Al Nile.ttc" on macOS.
f, err := ioutil.ReadFile(os.Args[1])
if err != nil {
return err
}
tt, err := truetype.Parse(f)
if err != nil {
return err
}
face := truetype.NewFace(tt, &truetype.Options{
Size: 16,
DPI: 72,
Hinting: font.HintingFull,
})
d := font.Drawer{
Dst: dst,
Src: image.NewUniform(color.Black),
Face: face,
Dot: fixed.P(ox, oy),
}
for _, l := range strings.Split(text, "\n") {
d.DrawString(l)
d.Dot.X = fixed.I(ox)
d.Dot.Y += face.Metrics().Height
}
path := "example.png"
fout, err := os.Create(path)
if err != nil {
return err
}
defer fout.Close()
if err := png.Encode(fout, d.Dst); err != nil {
return err
}
if err := browser.OpenFile(path); err != nil {
return err
}
return nil
}
func main() {
flag.Parse()
if err := run(); err != nil {
panic(err)
}
}
```
### What did you expect to see?
The text is rendered correctly in an image.
### What did you see instead?
The text is rendered wrongly:

I was also wondering how to render texts in complex languages like Thai or Hindi.
| help wanted,NeedsInvestigation,FeatureRequest | low | Critical |
354,415,753 | rust | Missed unused import in 2018 edition when importing a crate | Code like this:
```rust
fn main() {
test1::foo();
test2::foo();
}
mod test1 {
use std;
pub fn foo() {
std::mem::drop(3);
}
}
mod test2 {
use std;
pub fn foo() {
std::println!("x");
}
}
```
[when compiled](https://play.rust-lang.org/?gist=b78735ca06dda3b786c653f9ca3edbdf&version=nightly&mode=debug&edition=2018) yields no warnings currently. In theory, though, both imports of `std` are unused due to the 2018 crate prelude! | C-enhancement,A-lints,T-compiler,A-edition-2018 | low | Critical |
354,433,589 | godot | Newly created built-in GDScript can point to existing script or back to discarded built-in script. | Working from Godot 3.1, 64-bit Windows, 8/27/2018
## Update - Animated GIF
Points to existing script with similar name, until toggling the built-in option button. Then points to the same built-in script over and over.
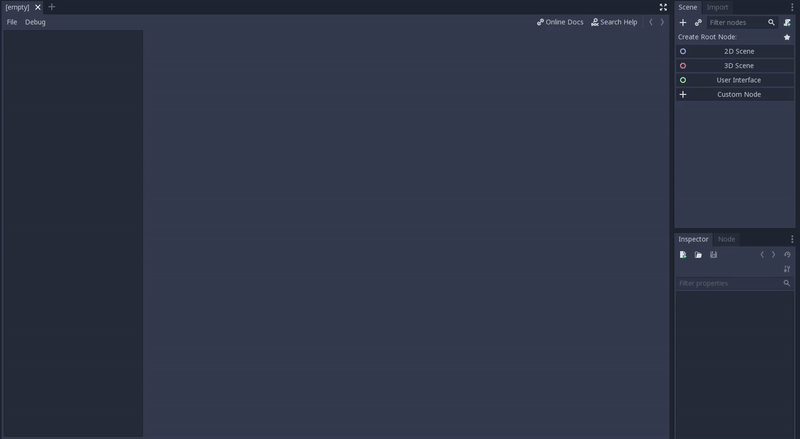
Have been noticing that using built-in scripts have been having a terrible time of pointing back to some other script, instead of making a new script. And the only way to fix this is to manually clear it, and then forcefully close a certain unnamed script in the script editor.
Steps to reproduce. (From a new project)
1. Set empty/unsaved scene's root node. Then attach a script and choose "Built-in Script".
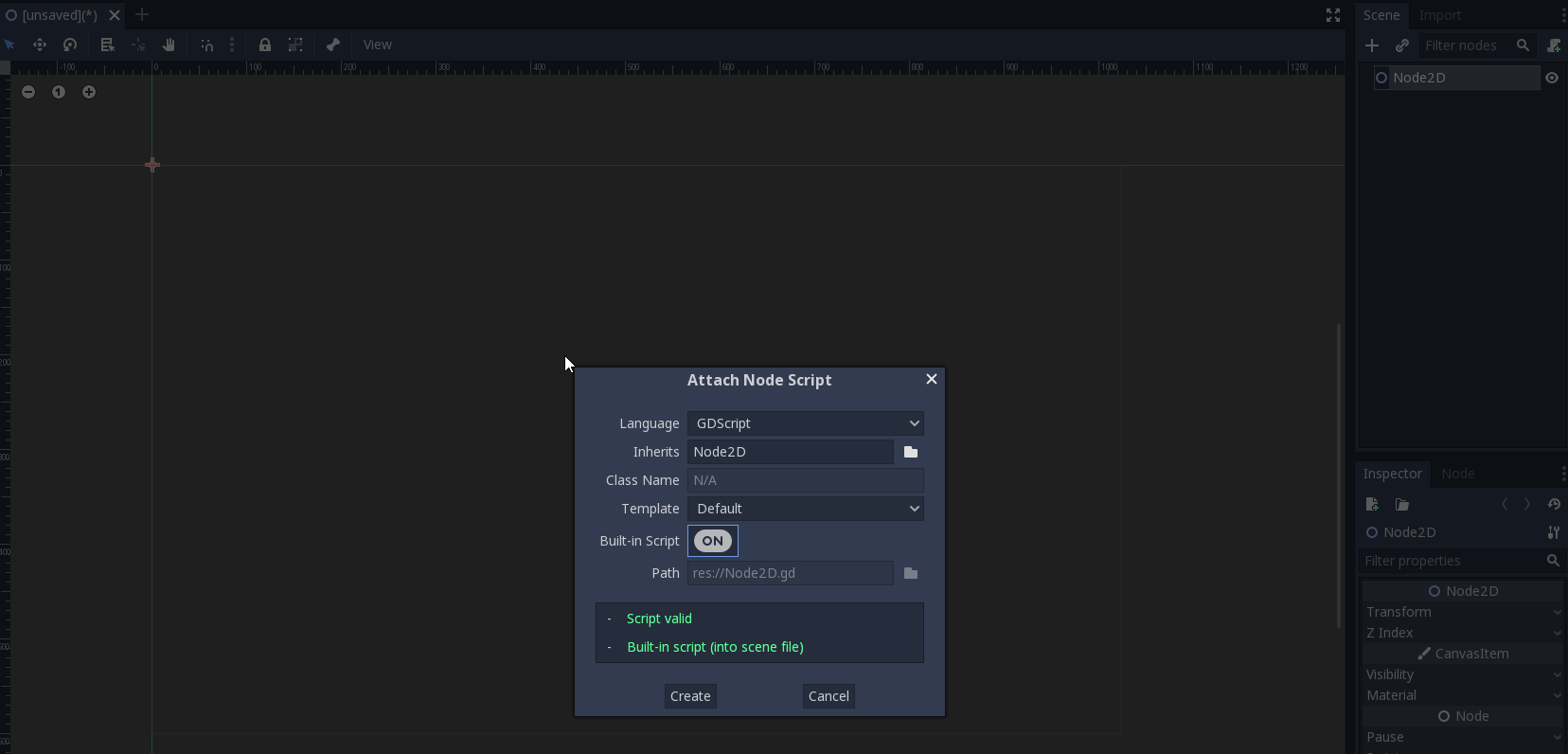
2. Type some code that isn't the default generated script. (Otherwise it won't be obvious what is happens next.)
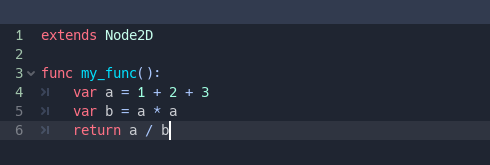
3. Close that scene without saving it.
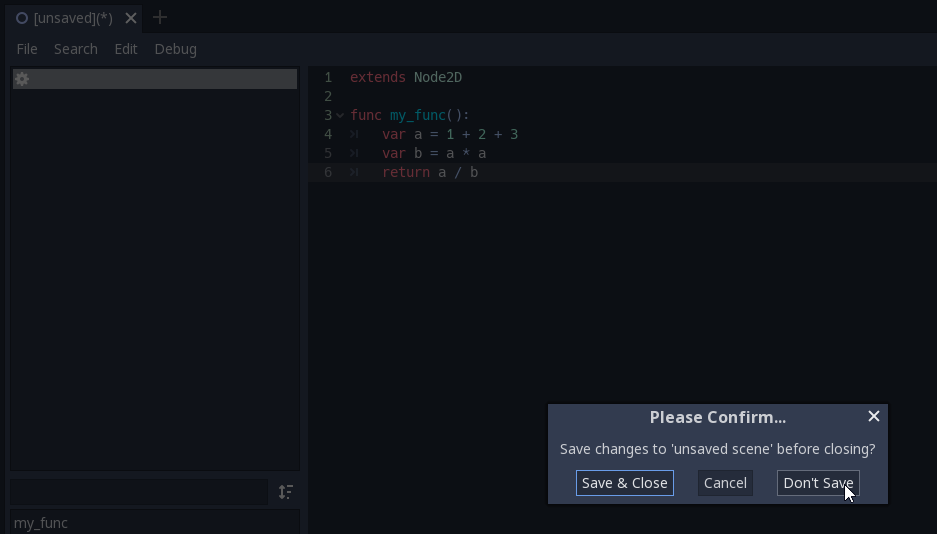
4. In the empty tab, start again, by setting up a root node, then add another "Built-in Script".

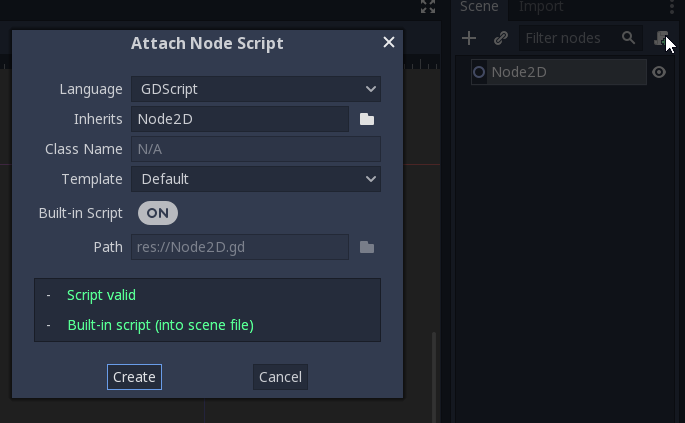
5. Now your new built-in script seems to be that unnamed ghost script floating about.

6. After carrying on like this, each new one you try is going to do this, until something is done to get rid of this unnamed script.
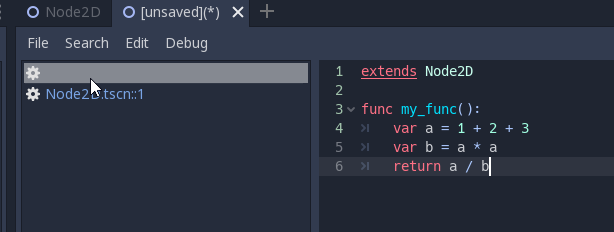
Perhaps worse still.
If you have a typo and mangle the name of what the script `extends`, you'll get stuck with a message like below. Then anytime you try to add a new script in the hopes of quickly cleaning it up by overwriting it, it will not allow it, and have a disabled Create button. Even with an external script option.
It takes a fair bit of manual clearing and cleaning up to rectify it.
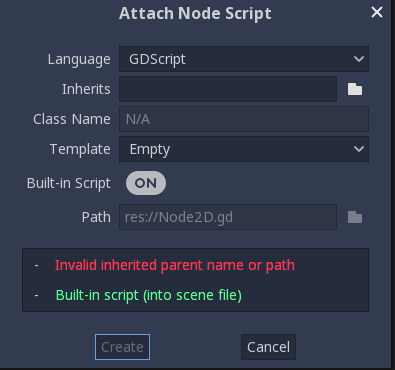
| bug,topic:editor,confirmed | low | Minor |
354,434,166 | flutter | Suggestion: Add stream bindable constructors to allow widgets to automatically update | Using a `bloc` pattern in combination with `StreamBuilder` results in a responsive UI, but the builder code ends up being all over the place. What if we had a new constructor on some widgets, such as `Text` that could have this function internally.
`const Text.bound(this.stream, ...);`
It could work similar to how this Widget is structured:
``` dart
import 'dart:async';
import 'package:flutter/material.dart';
class BoundText extends StatelessWidget {
BoundText(
this.stream, {
this.style,
});
final Stream<dynamic> stream;
final TextStyle style;
@override
Widget build(BuildContext context) {
return StreamBuilder(
stream: stream,
builder: (content, snapshot) {
return Text(snapshot.data.toString(), style: style);
},
);
}
}
```
| c: new feature,framework,P2,team-framework,triaged-framework | low | Minor |
354,438,220 | go | gccgo: GOPATH=/opt/go_pkgs go get -v golang.org/x/sys/... fails | ### What version of Go are you using (`go version`)?
`go version go1.10.3 gccgo (GCC) 8.2.1 20180813 solaris/sparc
`
### Does this issue reproduce with the latest release?
`yes (gccgo compiled from source)
`
### What operating system and processor architecture are you using (`go env`)?
```
amandeep@s113ldom1:~/workspace/main$ ago env
-bash: ago: command not found
amandeep@s113ldom1:~/workspace/main$ ago ^C
amandeep@s113ldom1:~/workspace/main$ go env
GOARCH="sparc"
GOBIN=""
GOCACHE="/home/amandeep/.cache/go-build"
GOEXE=""
GOHOSTARCH="sparc"
GOHOSTOS="solaris"
GOOS="solaris"
GOPATH="/opt/go_pkgs/"
GORACE=""
GOROOT="/usr/gnu"
GOTMPDIR=""
GOTOOLDIR="/usr/gnu/libexec/gcc/sparc-sun-solaris2.11/8.2.1"
GCCGO="/usr/gnu/bin/gccgo"
CC="gcc"
CXX="g++"
CGO_ENABLED="1"
CGO_CFLAGS="-g -O2"
CGO_CPPFLAGS=""
CGO_CXXFLAGS="-g -O2"
CGO_FFLAGS="-g -O2"
CGO_LDFLAGS="-g -O2"
PKG_CONFIG="pkg-config"
GOGCCFLAGS="-fPIC -pthread -fmessage-length=0 -fdebug-prefix-map=/tmp/go-build275813452=/tmp/go-build -gno-record-gcc-switches -funwind-tables"
```
### What did you do?
`sudo GOPATH=/opt/go_pkgs go get -v golang.org/x/sys/...`
### What did you expect to see?
package to install
### What did you see instead?
this is how the prompt looks:
```
amandeep@s113ldom1:~/workspace/main$ sudo GOPATH=/opt/go_pkgs go get -v golang.org/x/sys/...
golang.org/x/sys/cpu
golang.org/x/sys/windows
golang.org/x/sys/unix
golang.org/x/sys/windows/registry
# golang.org/x/sys/cpu
/opt/go_pkgs/src/golang.org/x/sys/cpu/cpu.go:10:30: error: reference to undefined name ‘cacheLineSize’
type CacheLinePad struct{ _ [cacheLineSize]byte }
^
# golang.org/x/sys/unix
/opt/go_pkgs/src/golang.org/x/sys/unix/sockcmsg_unix.go:15:12: error: reference to undefined name ‘sizeofPtr’
salign := sizeofPtr
^
/opt/go_pkgs/src/golang.org/x/sys/unix/syscall_unix.go:25:47: error: reference to undefined name ‘sizeofPtr’
darwin64Bit = runtime.GOOS == "darwin" && sizeofPtr == 8
^
/opt/go_pkgs/src/golang.org/x/sys/unix/syscall_unix.go:26:50: error: reference to undefined name ‘sizeofPtr’
dragonfly64Bit = runtime.GOOS == "dragonfly" && sizeofPtr == 8
^
/opt/go_pkgs/src/golang.org/x/sys/unix/syscall_unix.go:28:48: error: reference to undefined name ‘sizeofPtr’
solaris64Bit = runtime.GOOS == "solaris" && sizeofPtr == 8
^
/opt/go_pkgs/src/golang.org/x/sys/unix/sockcmsg_unix.go:28:21: error: reference to undefined name ‘SizeofCmsghdr’
return cmsgAlignOf(SizeofCmsghdr) + datalen
^
/opt/go_pkgs/src/golang.org/x/sys/unix/sockcmsg_unix.go:34:21: error: reference to undefined name ‘SizeofCmsghdr’
return cmsgAlignOf(SizeofCmsghdr) + cmsgAlignOf(datalen)
^
/opt/go_pkgs/src/golang.org/x/sys/unix/sockcmsg_unix.go:38:73: error: reference to undefined name ‘SizeofCmsghdr’
return unsafe.Pointer(uintptr(unsafe.Pointer(h)) + uintptr(cmsgAlignOf(SizeofCmsghdr)))
^
/opt/go_pkgs/src/golang.org/x/sys/unix/sockcmsg_unix.go:37:18: error: use of undefined type ‘Cmsghdr’
func cmsgData(h *Cmsghdr) unsafe.Pointer {
^
/opt/go_pkgs/src/golang.org/x/sys/unix/sockcmsg_unix.go:59:25: error: reference to field ‘Len’ in object which has no fields or methods
i += cmsgAlignOf(int(h.Len))
^
/opt/go_pkgs/src/golang.org/x/sys/unix/sockcmsg_unix.go:65:9: error: reference to undefined name ‘Cmsghdr’
h := (*Cmsghdr)(unsafe.Pointer(&b[0]))
^
/opt/go_pkgs/src/golang.org/x/sys/unix/sockcmsg_unix.go:65:8: error: expected pointer
h := (*Cmsghdr)(unsafe.Pointer(&b[0]))
^
/opt/go_pkgs/src/golang.org/x/sys/unix/sockcmsg_unix.go:66:13: error: reference to undefined name ‘SizeofCmsghdr’
if h.Len < SizeofCmsghdr || uint64(h.Len) > uint64(len(b)) {
^
/opt/go_pkgs/src/golang.org/x/sys/unix/sockcmsg_unix.go:67:20: error: reference to undefined name ‘EINVAL’
return nil, nil, EINVAL
^
/opt/go_pkgs/src/golang.org/x/sys/unix/sockcmsg_unix.go:69:26: error: reference to undefined name ‘SizeofCmsghdr’
return h, b[cmsgAlignOf(SizeofCmsghdr):h.Len], nil
and many more......
```
| OS-Solaris,NeedsFix | low | Critical |
354,447,383 | godot | Project loses its default environment configuration | <!-- Please search existing issues for potential duplicates before filing yours:
https://github.com/godotengine/godot/issues?q=is%3Aissue
-->
**Godot version:**
<!-- Specify commit hash if non-official. -->
current tip
0565adb20ff01a758c5ae8e4534a2cb7f07952df
**OS/device including version:**
<!-- Specify GPU model and drivers if graphics-related. -->
Linux
**Issue description:**
<!-- What happened, and what was expected. -->
Open a project with a **textured panorama-sky** environment:
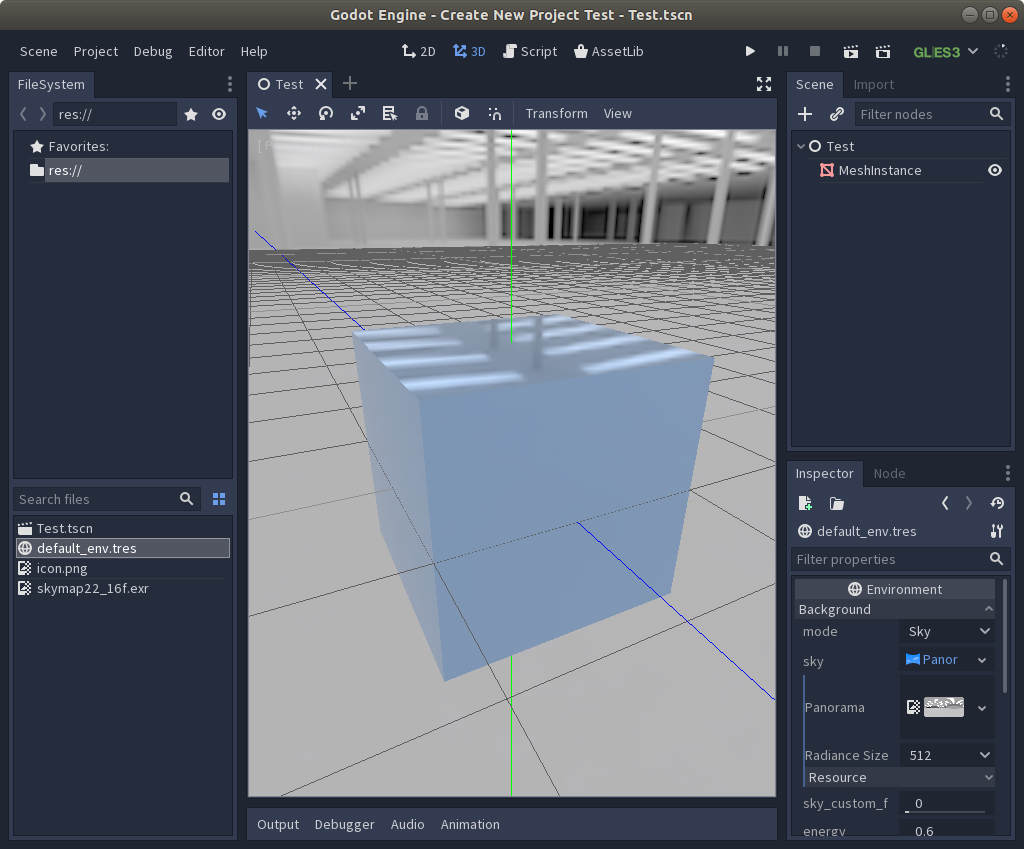
```
; Engine configuration file.
; It's best edited using the editor UI and not directly,
; since the parameters that go here are not all obvious.
;
; Format:
; [section] ; section goes between []
; param=value ; assign values to parameters
config_version=4
_global_script_classes=[ ]
_global_script_class_icons={
}
[application]
config/name="Create New Project Test"
run/main_scene="res://Test.tscn"
config/icon="res://icon.png"
[rendering]
environment/default_environment="res://default_env.tres"
```
Close the editor and remove the cached files (I usually have .import/ in .gitignore) :
**rm -rf .import/**
Start the editor again:
**godot3 -e**
```OpenGL ES 3.0 Renderer: AMD Radeon HD 7700 Series (CAPE VERDE / DRM 3.26.0 / 4.18.1-041801-generic, LLVM 6.0.0)
ERROR: _load_data: Condition ' !f ' is true. returned: ERR_CANT_OPEN
At: scene/resources/texture.cpp:469.
ERROR: _load: Failed loading resource: res://.import/skymap22_16f.exr-ce9b31538453c1bf4ce871fe24de66de.stex
At: core/io/resource_loader.cpp:190.
ERROR: _load: Failed loading resource: res://skymap22_16f.exr
At: core/io/resource_loader.cpp:190.
ERROR: poll: res://default_env.tres:3 - Parse Error: [ext_resource] referenced nonexistent resource at: res://skymap22_16f.exr
At: scene/resources/scene_format_text.cpp:439.
ERROR: load: Condition ' err != OK ' is true. returned: RES()
At: core/io/resource_loader.cpp:153.
ERROR: _load: Failed loading resource: res://default_env.tres
At: core/io/resource_loader.cpp:190.
WARNING: cleanup: ObjectDB Instances still exist!
At: core/object.cpp:2081.
```
```; Engine configuration file.
; It's best edited using the editor UI and not directly,
; since the parameters that go here are not all obvious.
;
; Format:
; [section] ; section goes between []
; param=value ; assign values to parameters
config_version=4
_global_script_classes=[ ]
_global_script_class_icons={
}
[application]
config/name="Create New Project Test"
run/main_scene="res://Test.tscn"
config/icon="res://icon.png"
```
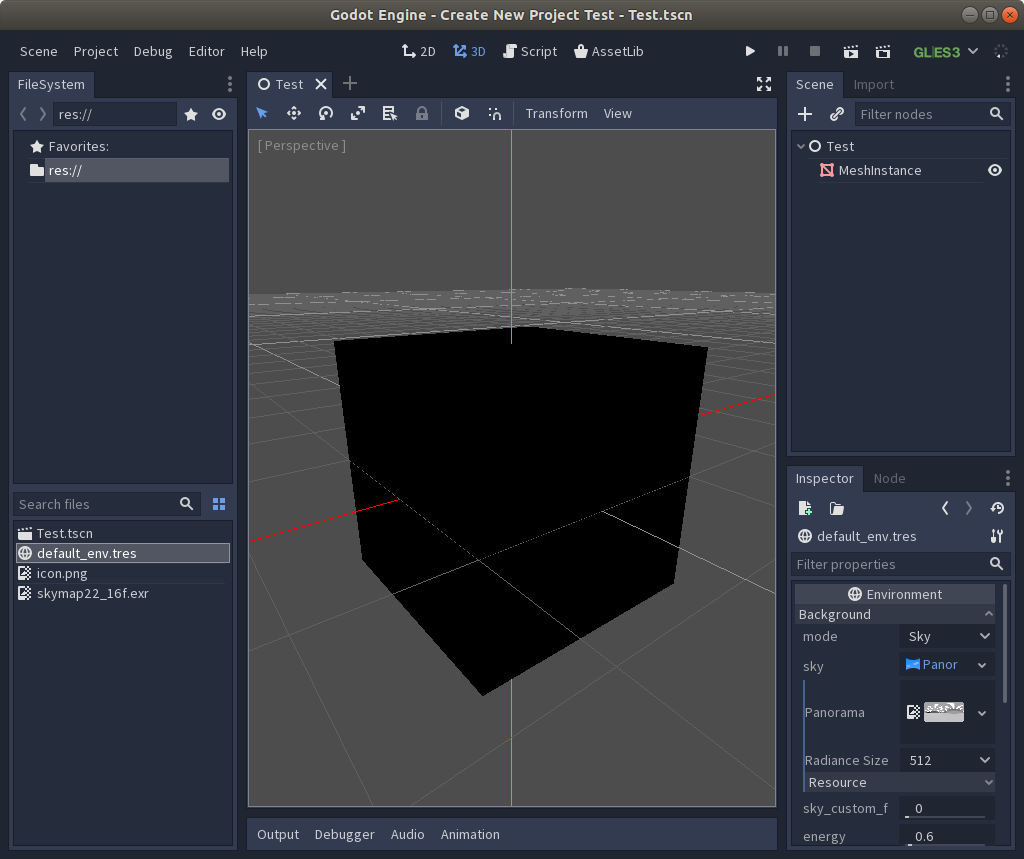
**Minimal reproduction project:**
<!-- Recommended as it greatly speeds up debugging. Drag and drop a zip archive to upload it. -->
[default-env-test.zip](https://github.com/godotengine/godot/files/2325372/default-env-test.zip)
| bug,topic:editor,confirmed,topic:3d | low | Critical |
354,468,258 | pytorch | Cannot find operator schema for 'ATen' Caffe2 Ios | ## Issue description
Running a network with ATen ops on caffe2 built for Ios results in the following error message
## Error Message
```
[E operator.cc:140] Cannot find operator schema for ATen. Will skip schema checking.
libc++abi.dylib: terminating with uncaught exception of type at::Error: [enforce fail at operator.cc:209] op.
Cannot create operator of type 'ATen' on the device 'CPU'. Verify that implementation for the corresponding device exist.
It might also happen if the binary is not linked with the operator implementation code.
If Python frontend is used it might happen if dyndep.InitOpsLibrary call is missing.
Operator def: input: "150" output: "151" output: "152" name: "" type: "ATen" arg { name: "dim" i: 1 } arg { name: "keepdim" i: 0 } arg { name: "operator" s: "max" } device_option { device_type: 0 cuda_gpu_id: 0 }
```
## System Info
- Caffe2
- Installed from Source ([Instructions followed](https://caffe2.ai/docs/getting-started.html?platform=ios&configuration=compile))
- Additional build command: -DBUILD_ATEN_MOBILE=ON
- OS: macOS 10.13.6
- CMake version: 3.12.1
## More Information
The model was written and trained in pytorch. The onnx export file and init_net.pb as well as predict_net.pb files were generated by following the [Transfering a model from PyTorch to Caffe2 and Mobile using ONNX](https://pytorch.org/tutorials/advanced/super_resolution_with_caffe2.html#transfering-a-model-from-pytorch-to-caffe2-and-mobile-using-onnx) tutorial
Superresolution Net and Squeeze Net both execute without fail. The error message mentions linking the operator implementation code. I'm not sure how to go about that in my XCode project
| caffe2,triaged | low | Critical |
354,490,712 | rust | Compiler runs out of memory | I've trying to change a crate to be more generic, so I've started to add some generics to some types and then continued to add them where the compiler suggests (more or less).
That worked pretty fine till the crate stopped "compiling" because it is running out of memory (with a peak of ~30GB) and gets killed by the OS.
The code the reproduce this is unfortunately [quite big](https://github.com/weiznich/juniper/commit/21ed21506d270ebe4d576e29773fe5f17064a8c0) because I don't know what exactly caused this.
There are not that much generics in there, compared to for example diesel, so I don't think the generics itself are the problem. More likely this is the result of a half finished transformation of everything to the new generic form.
This happens with the latest rustc nightly. | T-compiler,I-compilemem,C-bug,E-needs-mcve | low | Major |
354,529,736 | vue | Vue.component doesnt except argument of type `Component` | ### Version
2.5.17
### Reproduction link
[https://codesandbox.io/s/8z04jxj3y8](https://codesandbox.io/s/8z04jxj3y8)
### Steps to reproduce
- Click repro link
- Open test.ts
- See type errors
- Uncomment vue type augmentation to see errors disappear
### What is expected?
I can pass any component to `Vue.component`
### What is actually happening?
Type error:
```
Argument of type 'Component<DefaultData<never>, DefaultMethods<never>, DefaultComputed, Record<string, any>>' is not assignable to parameter of type 'ComponentOptions<Vue, DefaultData<Vue>, DefaultMethods<Vue>, DefaultComputed, PropsDefinition<Rec...'.
Type 'VueConstructor<Vue>' is not assignable to type 'ComponentOptions<Vue, DefaultData<Vue>, DefaultMethods<Vue>, DefaultComputed, PropsDefinition<Rec...'.
Value of type 'VueConstructor<Vue>' has no properties in common with type 'ComponentOptions<Vue, DefaultData<Vue>, DefaultMethods<Vue>, DefaultComputed, PropsDefinition<Rec...'. Did you mean to call it?
```
---
Adding the following overload to `Vue.component` will fix it:
```ts
component<Data, Methods, Computed, Props>(
id: string,
definition: Component<Data, Methods, Computed, Props>,
): ExtendedVue<V, Data, Methods, Computed, Props>;
```
<!-- generated by vue-issues. DO NOT REMOVE --> | typescript | low | Critical |
354,530,770 | flutter | flutter_test framework does not run isolates/handle isolate message sending properly | Isolates are unable to send and receive messages properly when running flutter_test tests. For example, even very simple ping-pong style messages either fail to send or the isolate may fail to run at all.
```dart
import 'dart:isolate';
import 'package:unittest/unittest.dart';
child(args) {
var msg = args[0];
var reply = args[1];
print("msg = $msg, reply = $reply");
reply.send('re: $msg');
}
void main([args, port]) {
test('message - reply chain', () {
ReceivePort port = new ReceivePort();
port.listen((msg) {
print("Received msg + $msg");
port.close();
});
Isolate.spawn(child, ['hi', port.sendPort]);
});
}
```
This simple example shows one of the symptoms of the issue where the 're: $msg' is never printed.
Other examples sometimes run the child isolate code, but the reply messages to the reply port fails to send. | a: tests,framework,dependency: dart,has reproducible steps,P2,found in release: 3.5,team-framework,triaged-framework | low | Major |
354,553,306 | rust | Builtin macros such as `include_str!` should accept `&'static str` instead of a string literal | Right now, `include_str!()` works on a syntax level and does not allow a user to pass in a `&'static str` for the file path. I stumbled upon this after the following code didn't compile in release mode:
```rust
const CSS_PATH: &str = "C:\\my\\file\\path\\test.css";
#[cfg(debug_assertions)]
let css = Css::hot_reload(CSS_PATH).unwrap();
#[cfg(not(debug_assertions))]
let css = Css::new_from_str(include_str!(CSS_PATH)).unwrap();
```
Notice the `Css::hot_reload` vs the `Css::new_from_str` - in debug mode I want to hot-reload the file from disk (for quick style changes), but in release mode I want to include it in the binary.
Now I can solve it by doing:
```rust
#[cfg(debug_assertions)]
let css = Css::hot_reload("C:\\my\\file\\path\\test.css").unwrap();
#[cfg(not(debug_assertions))]
let css = Css::new_from_str(include_str!("C:\\my\\file\\path\\test.css")).unwrap();
```
... but then I need to update the file path in two places, which isn't good.
Looking at the [source of `include_str!()`](https://github.com/rust-lang/rust/blob/727eabd68143e968d8826ee29b8ea7792d29fa96/src/libsyntax/ext/source_util.rs#L133-L168), it seems that `include_str!` works directly on the AST, i.e. the compiler doesn't know about whether the given string (the file path) is `const` or not, so if it is implemented it would maybe be necessary to restructure this part of the compiler.
I realize that it's a low-priority issue, but it would be nice to have. And yes, I can solve it with a build script, but that's just a last line of defense - I currently don't see any reason (except from the technical implementation problem) to not have it in the compiler - maybe a potential security concern if the strings are built at compile time from a `const fn`, but then again `include_str!("/usr/bin/passwd")` can already be done today, so it doesn't decrease or increase security. | T-compiler,C-feature-request,A-const-eval | low | Critical |
354,604,329 | go | x/mobile/cmd/gomobile: bind fails with interface syntax | Please answer these questions before submitting your issue. Thanks!
### What version of Go are you using (`go version`)?
go version go1.11 darwin/amd64
gomobile version +bceb7ef Wed Aug 8 22:10:59 2018 +0000 (android,ios); androidSDK=
### Does this issue reproduce with the latest release?
yes
### What operating system and processor architecture are you using (`go env`)?
GOARCH="amd64"
GOBIN=""
GOCACHE="/Users/michael/Library/Caches/go-build"
GOEXE=""
GOFLAGS=""
GOHOSTARCH="amd64"
GOHOSTOS="darwin"
GOOS="darwin"
GOPATH="/Users/michael/Projects/golang"
GOPROXY=""
GORACE=""
GOROOT="/usr/local/Cellar/go/1.11/libexec"
GOTMPDIR=""
GOTOOLDIR="/usr/local/Cellar/go/1.11/libexec/pkg/tool/darwin_amd64"
GCCGO="gccgo"
CC="clang"
CXX="clang++"
CGO_ENABLED="1"
GOMOD=""
CGO_CFLAGS="-g -O2"
CGO_CPPFLAGS=""
CGO_CXXFLAGS="-g -O2"
CGO_FFLAGS="-g -O2"
CGO_LDFLAGS="-g -O2"
PKG_CONFIG="pkg-config"
GOGCCFLAGS="-fPIC -m64 -pthread -fno-caret-diagnostics -Qunused-arguments -fmessage-length=0 -fdebug-prefix-map=/var/folders/bd/rnhtmc553vvcm_ty8nt2tv4c0000gn/T/go-build433537712=/tmp/go-build -gno-record-gcc-switches -fno-common"
### What did you do?
I'm try to build [v2ray](https://github.com/v2ray/v2ray-core/) for ios app.
``` shell
gomobile bind -target=ios v2ray.com/core
```
fail:
```
gomobile: darwin-arm: go build -tags ios -buildmode=c-archive -o /var/folders/bd/rnhtmc553vvcm_ty8nt2tv4c0000gn/T/gomobile-work-288847091/core-arm.a gobind failed: exit status 2
# gobind
/var/folders/bd/rnhtmc553vvcm_ty8nt2tv4c0000gn/T/gomobile-work-288847091/src/gobind/go_coremain.go:1258:9: cannot use (*proxycore_OutboundHandler)(_res_ref) (type *proxycore_OutboundHandler) as type core.OutboundHandler in assignment:
*proxycore_OutboundHandler does not implement core.OutboundHandler (missing Dispatch method)
/var/folders/bd/rnhtmc553vvcm_ty8nt2tv4c0000gn/T/gomobile-work-288847091/src/gobind/go_coremain.go:1273:9: cannot use (*proxycore_OutboundHandler)(_res_ref) (type *proxycore_OutboundHandler) as type core.OutboundHandler in assignment:
*proxycore_OutboundHandler does not implement core.OutboundHandler (missing Dispatch method)
```
a simplified case like this
``` go
package hello
//import "context"
//
//type A interface {
// Run(ctx context.Context)
//}
//
//type BB interface {
// GetA() A
//}
//------- or -------
type C bool
type A interface {
AA() C
}
type BB interface {
AAA() A
}
```
``` shell
gomobile bind -target=ios v2ray.com/core/hello
```
result
```
gomobile: darwin-arm: go build -tags ios -buildmode=c-archive -o /var/folders/bd/rnhtmc553vvcm_ty8nt2tv4c0000gn/T/gomobile-work-922396824/hello-arm.a gobind failed: exit status 2
# gobind
/var/folders/bd/rnhtmc553vvcm_ty8nt2tv4c0000gn/T/gomobile-work-922396824/src/gobind/go_hellomain.go:55:9: cannot use (*proxyhello_A)(_res_ref) (type *proxyhello_A) as type hello.A in assignment:
*proxyhello_A does not implement hello.A (missing AA method)
```
### What did you expect to see?
Success build
| NeedsInvestigation,mobile | low | Critical |
354,612,640 | vscode | Settings UI lacks concept of inherited default value | re #57304
* open settings UI
* locate 'Suggest Font Size'
* notice how the UI shows `0` which isn't true - the default value is the font size of the editor.
<img width="343" alt="screen shot 2018-08-28 at 09 47 01" src="https://user-images.githubusercontent.com/1794099/44708754-32e69500-aaa8-11e8-9088-194a04f4d6bc.png">
| feature-request,settings-editor | low | Major |
354,659,264 | vscode | Settings decoration: Modified in Folder | Issue Type: <b>Feature Request</b>
Testing #57304
There already is a 'Modified in Workspace" decoration, it would be great if that would also list any folders in which that setting is modified (and also show these in the workspace settings).
VS Code version: Code - Insiders 1.27.0-insider (43676d0cb7db94e2fa771f1d10bff10eaa01b453, 2018-08-28T05:12:24.162Z)
OS version: Darwin x64 17.7.0
<!-- generated by issue reporter --> | feature-request,settings-editor | low | Minor |
354,661,133 | pytorch | [Caffe2] Model work Alright in Python but Failed in C++ | ## Issue description
I have built a model in PyTorch and transfer it into Caffe2's .pb format through onnx. The model in .pb format worked well with Caffe2's Python API, but failed to work with Caffe2's C++ API. I rewrote the C++ code following the pybind_state.cc file in my local repository of caffe2 source code, making my C++ code almost identical to the reference. However, it still didn't work. My guess is that the problem lies in the caffe2.pb.cc file generated using protobuf, as I generated it after my build of Caffe2 following the tutorial in https://caffe2.ai/docs/cplusplus_tutorial.html.
## Code example
Following is my C++ code.
```
this->workspace = new caffe2::Workspace();
caffe2::NetDef initNet, predictNet;
CAFFE_ENFORCE(this->workspace);
std::ifstream initNetFile(this->initNetPath, ios::binary);
std::ifstream predictNetFile(this->predictNetPath, ios::binary);
std::string initNetStr((std::istreambuf_iterator<char>(initNetFile)), std::istreambuf_iterator<char>());
std::string predictNetStr((std::istreambuf_iterator<char>(predictNetFile)), std::istreambuf_iterator<char>());
CAFFE_ENFORCE(caffe2::ParseProtoFromLargeString(initNetStr, &initNet));
CAFFE_ENFORCE(caffe2::ParseProtoFromLargeString(predictNetStr, &predictNet));
auto predictor = new caffe2::Predictor(initNet, predictNet, this->workspace);
```
And my Python demo which worked.
```
# The path to save model
INIT_MODEL_PATH = r".\init_voxelbased.pb"
PREDICT_MODEL_PATH = r".\predict_voxelbased.pb"
# Load the model
with open(INIT_MODEL_PATH, 'rb') as f:
init_net = f.read()
with open(PREDICT_MODEL_PATH, 'rb') as f:
predict_net = f.read()
# Initialize the predictor from the input protobufs
p = workspace.Predictor(init_net, predict_net)
```
Visual Studio 2017 shows very little information (internal metadata point to 0x00000000000) about 'initNet' after the 'ParseProtoFromLargeString', so I strong suspect that there is something wrong with 'ParseProtoFromLargeString' .
## System Info
- PyTorch or Caffe2: Caffe2
- How you installed Caffe2 (conda, pip, source): source
- Build command you used (if compiling from source): build_windows.bat in '/scripts'
- OS: Windows 10 professional
- Caffe2 version: Not sure, but built from source in Aug 15 2018
- Python version: 3.6.5
- CUDA/cuDNN version: 9.2
- GPU models and configuration: GTX1080
- GCC version (if compiling from source): Unknown
- CMake version: 3.12.1
- Versions of any other relevant libraries: protobuf 3.5.0
| caffe2 | low | Critical |
354,664,338 | vue | Property 'xxx' does not exist on type CombinedVueInstance ? | ### Version
2.5.17
### Reproduction link
### Steps to reproduce
1. use vue-cli init a ts hello-world project .
2. code like that
```js
<script lang="ts">
import Vue from "vue";
export default Vue.extend({
name: "HelloWorld",
props: {
msg: String
},
data():any {
return {
wtf: this.initData(), // throw ts error : Property 'initData' does not exist on type 'CombinedVueInstance<Vue, {}, {}, {}, Readonly<{ msg: string; }>>'.
}
},
methods: {
initData():any {
return {
a: ''
}
},
},
});
</script>
```
### What is expected?
How can i fix it ?
### What is actually happening?
**Property 'initData' does not exist on type 'CombinedVueInstance<Vue, {}, {}, {}, Readonly<{ msg: string; }>>'.**
<!-- generated by vue-issues. DO NOT REMOVE --> | typescript | high | Critical |
354,697,447 | godot | moving folded code only moves top line from fold and not all lines in fold |
Godot 3.1
usinmg alt+arrows to move a folded function over another folded function but only moved the top line from the folded function
unexpected behavior
make a function, fold it, use alt+arrows up or down to move it
| bug,topic:editor,confirmed | low | Minor |
354,697,758 | react | Number input breaks when letter "e" is entered | <!--
Note: if the issue is about documentation or the website, please file it at:
https://github.com/reactjs/reactjs.org/issues/new
-->
**Do you want to request a *feature* or report a *bug*?**
bug
**What is the current behavior?**
input[type=number] only allows entering numbers and letter "e" in the input. Native "input" input event is called for both numbers and the letter "e". With React the `onChange` event is only called for numbers. There's no way to catch "e" with `onChange`.
The "e" is even being filled when the input is controlled. The only way I can think of to work around this bug right now is to use `onKeyDown` and `preventDefault` when "e" or "E" is pressed.
**If the current behavior is a bug, please provide the steps to reproduce and if possible a minimal demo of the problem. Your bug will get fixed much faster if we can run your code and it doesn't have dependencies other than React. Paste the link to your JSFiddle (https://jsfiddle.net/Luktwrdm/) or CodeSandbox (https://codesandbox.io/s/new) example below:**
https://codesandbox.io/s/ov3ql3ljwz
**What is the expected behavior?**
It should pass anything that is being filled into the input to the onChange handler and should not break controlled component.
**Which versions of React, and which browser / OS are affected by this issue? Did this work in previous versions of React?**
React: 16.4.2
Chrome: 68.0.3440.106
Windows 10 | Component: DOM,Type: Needs Investigation | medium | Critical |
354,711,592 | rust | thinlto run-pass unit test link errors on OS X (High Sierra 10.13.6) | I have been getting errors in our run-pass test suite on OS X for some amount of time. I'm not sure if the problem is in a malconstructed `ld` invocation, or something else.
I'm posted a full transcript of the message from the `x.py test` run below in [this gist](https://gist.github.com/pnkfelix/8d01d8eaa3748a646847b93fc5ef435c), but here is probably the most relevant snippet of that output:
```
error: linking with `cc` failed: exit code: 1
|
= note: "cc" "-m64" "-L" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/stage2/lib/rustlib/x86_64-apple-darwin/lib" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.11dinsfm25j7lpyc.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1329kli0ah0e260m.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.133ixqypxn7wz1o0.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.136nl6d4hw5n28j5.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.137av9lascg77nun.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.13mladucca3tu5yp.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.144fd7vd07hgcxth.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.144fd7vd07hgcxth.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.15p1jbcuqwtmc6z9.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.17wx4s8c1vg6fm1c.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.195l647wv7e0399t.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1anzl8n8xydut875.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1asp41fcvkq6heh6.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1crzh4eb9rk3gpt5.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1cwt7uar7mashsjs.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1cwt7uar7mashsjs.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1fv3zoqe0an8tnd1.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1genq0xk28rpyff8.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1ghu8tqlqgendytg.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1hssl9uhtcgmbb26.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1ivu43lqofbb7khb.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1j44fln0amyp7ew0.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1jq4bww9ndj35t7d.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1k5h59phnz8gmkbt.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1l5howcfxb8c0bnp.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1mc3swg7idq9qhil.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1mp7fv9i7zyyoxwr.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1mp7fv9i7zyyoxwr.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1mp7fv9i7zyyoxwr.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1nb70bnwggol4of8.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1ogs1k2x8rhcykru.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1ogs1k2x8rhcykru.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1onqll48mq38irsu.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1opt8i9w78mtc849.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1p6c1ywqev6xfbsh.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1pbmnd3fr37vjqv.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1px1dcphmyl8ly4y.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1sbqca1lrqcmzus3.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1tahgyx5y63hqzn4.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1teoqnbl8vixq0v0.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1txyrxp0koogw7hk.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1u5sabw4z4er7tk4.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1uew3iio0vn0vdkt.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1up221vmg4d8oo9e.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1vgjp35ivgpbyt01.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1vgpq7sdd90t3smz.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1vods0mul5ypk9ai.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1w2azuc8r7te8u14.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1w5g2b9ro2tcwwwj.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1w890836k9n1val4.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1wcntmqg6o6kvyev.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1x3ywlurom8il190.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1x8djs5n5j5w0msf.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1ydu1tnbrdn111zw.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1yit1lao62ukl1b.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1yit1lao62ukl1b.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1z4at8cat3mwyt9n.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.1zxshk5phl9uuxjf.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2014bw3gs9742u6o.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.21f8t6tw6eat60np.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.22r184dg6fqxiq0l.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.22scjrnt1fawm9y0.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.22scjrnt1fawm9y0.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.22skfdbfhl1o6ad4.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.22skfdbfhl1o6ad4.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.245bh82i5dxmh8un.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.24dlr5qbblagiqbw.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.24o8ipc6y78663ds.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.24o8ipc6y78663ds.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.25setqrdfrvrbeve.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.26uqaeh7k4heqig0.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.26xq21gatn3ko6ef.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.27bkf2sge2lhl7qf.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.27gt2i3xb8z47flz.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.28zwgrwdyg4gonu3.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.290a73ep39awpkys.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.291uq78w1qto0rh1.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.292bljff6aa0lj92.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2bfs6wsyejut20p7.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2ce1lfrc8vjkueuo.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2cu9758ondtvr73s.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2dtan03jhs3bzy6a.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2dtan03jhs3bzy6a.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2dtan03jhs3bzy6a.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2fk5n68iiusb2o2e.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2fk5n68iiusb2o2e.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2fsridnkk8n84ow3.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2fsridnkk8n84ow3.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2fwxbjjaaz6acfqf.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2g95hkgskdkb9n6w.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2hmm4ydv2gxqun69.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2jby6bi09hfotcs8.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2jby6bi09hfotcs8.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2kld7gwihxo3cjsh.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2lpv8rz97ntfx18y.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2lpv8rz97ntfx18y.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2lpv8rz97ntfx18y.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2mh7pdgct65ubnw4.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2mhuelmwamaqoj1p.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2mr3t9w3e9e4leny.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2npc7b1x8cxysrn5.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2npc7b1x8cxysrn5.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2o869aobo6me9afq.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2ouginl193xyigt9.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2pcrlchn4k62lkds.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2qbfnblp57ogc3ed.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2r6y1c5m1q9tvxl3.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2rcbcggo4g57ukpm.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2t5ne39o3ormq5in.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2uou06yioxwmxea1.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2upeh09mjkwv7yct.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2uw42lhep80a8p79.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2ux7fts72602o2la.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2xxo4d60igyl1k4k.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2yopimyjnqaciubw.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.2zpeypgxprlfvwvv.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.308nq882b10w19zo.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.31rrmtetnw4w6rzj.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.334py42l6gmlbf5l.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.334py42l6gmlbf5l.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.34i7zlsky42z4njr.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.34i7zlsky42z4njr.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.34odee45o5qrqnes.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.34rm4hnmumopfevg.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.34rm4hnmumopfevg.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3631xtsryxxx9wsw.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3642moqeoufimxjv.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.364rpww7yqw2yh4d.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.37nijbctzfyu3b2f.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.37nijbctzfyu3b2f.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.384rpa6ufpfpd4db.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.38xbp6znk6dg93k0.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.394ikyrkqe5oob00.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.39lcgrjgy0dcn1yu.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.39qo4evr61pdyzdv.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.39yjz1o97wwlm8wp.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3bmbisy342u2r8hu.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3bpr6vuzuh02mdhp.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3cqcapqbjx49jo28.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3djyektau6frihp3.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3duxvae4hzh81tvv.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3edul2geepzglsy1.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3edul2geepzglsy1.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3edul2geepzglsy1.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3ejea9v2xuhkmc28.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3ejj5ppnzsmiijlr.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3g1bk6rold8lpb27.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3g63xgzlgfkdvw3o.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3gm8pbj3edrfoyt.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3gufsfl4y5ppmus1.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3hr8064ylwju25vo.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3htli8zb5peb1zjv.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3idg1w30nfb4fshz.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3je4pnr0jmb4z9c0.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3jhukhep9ktqe69o.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3jhukhep9ktqe69o.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3jhukhep9ktqe69o.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3k9dbv18apdaospq.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3lq078tu0k9q7wvg.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3ltg9m5j2q7vure8.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3ox528pp4ukvz6yz.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3prr39n7p1phi56o.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3quzwmkpnqmn81wh.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3qwe9ffgdgy0fp09.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3r346lz1amyxue8.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3spv5z99lfv7126g.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3vcw9mlisdw3yoq.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3we86esfhb7o91c5.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3yobgj0ytfcf23wp.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3yopuo9cllqyp3h.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3z2d2u6dynkqyf9a.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.3zqxgijhbrucjpz5.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.437crk07yt7rzprc.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.44h32gx48e0ln7ts.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.44mxth8tyv7jyn9e.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.45fsep75djvq2rxr.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.46swg4dagh7j9krq.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.46tco66fnw4yy3zn.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.46yyxkuo270nc8nx.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4840ddmc8twgbio2.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4bvb81wwcpeolgou.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4cvy5lzjswmc091y.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4d0pxxiyzci15q8n.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4d4pgimmb53urk8j.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4egtc9dssvzbe6oe.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4er2qk86jd7kug22.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4eu69xvwwnuxndze.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4eu69xvwwnuxndze.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4fo0twx6ry86jiq0.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4g05q72ti9qglr8a.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4gbmrgrwt3v1frbu.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4grts061vd2pbyty.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4gtriin8gzy4i46s.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4gyjtpp7e1wxkvn9.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4j57z3io05cqhl9o.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4jbv74yhq06mur3d.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4k7v69wa234em6nd.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4kfjo2j77pefkaa0.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4kqhfsmtsmdb035r.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4l1bkddoqn0xlgvu.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4lyz5xlpt7jtoh84.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4n19xd6bpt4kqnl7.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4n71doj9o0xj06e9.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4nwc64slfsuu1xyz.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4o0gbvp3j9sicrt1.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4oq2os59y9mgqchg.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4qcc6n9knkl0vek3.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4qzjgajr32x58s7t.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4to04iijh4y1oasf.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4udmilqrvp8jvxdb.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4udmilqrvp8jvxdb.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4uyb26921jnnpgi9.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4vc25fhy87kfcma0.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4wanectmi5q2ots8.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4wpd3f2wneeuaowf.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4wz0yexuflln6q2s.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4xlq8sslgnstey3g.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4xlq8sslgnstey3g.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4xo5n2fqry26axq7.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4z0g3xz0e1jgbiba.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4z2n17lq3tj9m4mk.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4z2n17lq3tj9m4mk.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.4zox0nqbm8npbq0m.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.50jfgi729foyz0db.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.511j279k1pt4pgzf.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.51hd4ctx20xfnwhb.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.51hd4ctx20xfnwhb.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.51hd4ctx20xfnwhb.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.52bnd7t94u1qylzv.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.52kzamnyg69ow5jg.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.545gdfdn9mds3att.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.54amskb2yfchlf.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.5695st4yy0b2cdq1.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.5822yu7fh8ziesh3.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.586mu6yuu0rj8d7a.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.58f2pmtlytnd6egv.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.59rbeehz75zw8zfn.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.5a92gxrr5f393b7u.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.5aw3pmtp1eqv9b5u.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.5cflvxgirer6x64x.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.5ctm0iioz9b8iv5p.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.5dck4im732ivkja.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.5e7srz8f6o00dr14.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.5e7srz8f6o00dr14.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.5e7srz8f6o00dr14.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.5ek3xp251j5yjehy.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.5eny8wdqgsskejkg.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.5fgps7cwto4ycdgu.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.5gjqxn4cu4xwix6k.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.5mvj9186s8ornbm.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.6njfqcsmf565fja.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.6njfqcsmf565fja.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.6njfqcsmf565fja.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.6njfqcsmf565fja.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.6oubks1jbizez33.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.6w89douk7f3bs64.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.7aqprds8vi0ni0e.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.7aqprds8vi0ni0e.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.7bd8sq3ib2798rk.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.7tz8dl9z1wmbdar.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.86fpkca4e00ps9r.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.8dyf5j1l3rc1b46.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.8rrw0wb8rs21mku.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.afeqmtho5vxji8u.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.all_crates.7rcbfp3g-cgu.0.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.all_crates.7rcbfp3g-cgu.1.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.c1mk16e6voenemm.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.czqc54ucerxw1i2.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.df9lv7isvh6ortb.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.dseg22bxfxrpqph.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.dt54n7tto61vp9n.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.end9egkogdg8gvn.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.es85ml4ueq8jivg.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.fqmxvlwp4o0tmwh.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.h2bn0okpognn8n5.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.hzjszg63gb0phx9.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.jlgsi6rbys2ncyd.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.jlgsi6rbys2ncyd.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.klpwrvd7yg9808u.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.kva8radzhm08rx7.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.kva8radzhm08rx7.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.kva8radzhm08rx7.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.lbjqm9bbbtfettz.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.ljcwis22olz2lxo.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.lmgzzvvvkblzo5t.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.n099l6ugv1zeyx.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.n8rpx71nz33ypfi.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.nvrv98cvo2g1g50.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.pwrase5dvtftia2.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.q54isor0o6fs5w5.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.qenypgync2j53qx.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.qhoe87t0fo0s7z0.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.raj22snkk31tcik.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.s07y03zix53fc8r.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.s1ep4j1t4nxduyr.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.s415d5v3bos2qej.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.szoydolxrmulqid.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.u3ocbviyehsoj31.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.u9z0780t7t4j66t.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.uozc6tpin9mgkfw.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.uozc6tpin9mgkfw.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.uvaxjxm49ruzhse.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.uvaxjxm49ruzhse.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.vq49yth2jl5tdp1.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.vv04s86xsf2i36d.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.wfy8o1llid1li54.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.wfy8o1llid1li54.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.xe0pisygxcurgg2.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.xe0pisygxcurgg2.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.y5ggzt25fds4jt.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.yt3h1s73ov94j67.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.zvp8kr56wi4wxic.rcgu.o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a.zx7mkldnttuzb0v.rcgu.o" "-o" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/a" "-Wl,-dead_strip" "-nodefaultlibs" "-L" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/native/rust-test-helpers" "-L" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/auxiliary" "-L" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/stage2/lib/rustlib/x86_64-apple-darwin/lib" "/var/folders/68/bxt927zn2tnbpj7qf4_4vpk80000gn/T/rustcQkyyRb/libstd-f599b8a2ea5b6d2a.rlib" "/var/folders/68/bxt927zn2tnbpj7qf4_4vpk80000gn/T/rustcQkyyRb/liballoc_jemalloc-2fe6902eecf8412f.rlib" "/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/stage2/lib/rustlib/x86_64-apple-darwin/lib/libcompiler_builtins-b5fd5c997086ce2a.rlib" "-lSystem" "-lresolv" "-lpthread" "-lc" "-lm" "-Wl,-rpath,@loader_path/../../../../stage2/lib/rustlib/x86_64-apple-darwin/lib" "-Wl,-rpath,/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/lib/rustlib/x86_64-apple-darwin/lib"
= note: ld: warning: directory not found for option '-L/Users/fklock/Dev/Mozilla/rust.git/objdir-dbgopt/build/x86_64-apple-darwin/test/run-pass/thinlto/all-crates/auxiliary'
Undefined symbols for architecture x86_64:
"_$LT$$RF$$u27$a$u20$T$u20$as$u20$core..fmt..UpperHex$GT$::fmt::h72b48078143e2674", referenced from:
std::sys_common::bytestring::debug_fmt_bytestring::h07191042f44f46b0 in a.y5ggzt25fds4jt.rcgu.o
"_$LT$alloc..vec..Vec$LT$T$GT$$GT$::drain::h8fe4e6bab243df27", referenced from:
_$LT$std..io..buffered..BufWriter$LT$W$GT$$GT$::flush_buf::hd937ab291f70bb51 in a.es85ml4ueq8jivg.rcgu.o
...
... [snipped ~350 lines of output] ...
...
_$LT$alloc..sync..Arc$LT$T$GT$$GT$::drop_slow::h78a65b9ea029d9fa in a.1vgjp35ivgpbyt01.rcgu.o
core::ptr::drop_in_place::hf68ceacf1d345d41 in a.1w5g2b9ro2tcwwwj.rcgu.o
_$LT$F$u20$as$u20$alloc..boxed..FnBox$LT$A$GT$$GT$::call_box::h3e18d1f71edfb8da in a.1w5g2b9ro2tcwwwj.rcgu.o
...
ld: symbol(s) not found for architecture x86_64
clang: error: linker command failed with exit code 1 (use -v to see invocation)
error: aborting due to previous error
```
it is annoying and I don't yet know when it started. I suppose I might attempt to bisect.
But I just wanted to make sure the problem was registered somewhere.
Oh, in case its relevant, here is my current `ld` and `xcode` version info:
```
% ld -v
@(#)PROGRAM:ld PROJECT:ld64-351.8
configured to support archs: armv6 armv7 armv7s arm64 i386 x86_64 x86_64h armv6m armv7k armv7m armv7em (tvOS)
LTO support using: LLVM version 9.1.0, (clang-902.0.39.2) (static support for 21, runtime is 21)
TAPI support using: Apple TAPI version 9.1.0 (tapi-902.0.9)
```
```
% xcodebuild -version
Xcode 9.4.1
Build version 9F2000
```
(Oh, and also: I am observing this for both stage 1 and stage 2 compilers.) | A-linkage,O-macos,T-compiler,C-bug | low | Critical |
354,756,511 | rust | rustdoc ignores inherent impls made in non-module scope | Similar to https://github.com/rust-lang/rust/issues/52545, rustdoc doesn't see anything if it's not written into a module's scope. However, there are some things that can be written in function/expression scope that still make it out into the public API. While that issue was about trait impls, it seems that inherent impls work in a similar way. Consider the following snippet:
```rust
fn asdf() {
impl SomeStruct {
pub fn qwop(&self) {
println!("hidden function");
}
}
}
fn main() {
let asdf = SomeStruct;
asdf.qwop(); // prints "hidden function"
}
```
Even though `qwop()` was written in function scope, it's still visible globally because it was defined with `pub`. Rustdoc should collect these impls and display them.
One thing i'm curious about is whether we should show non-`pub` functions defined in non-module scope when `--document-private-items` is set. I think we can get away with it, because it already displays private functions there regardless of where they're defined. | T-rustdoc,E-medium,C-bug | low | Minor |
354,786,889 | go | x/net/websocket: add a web socket client when targeting js/wasm | Please answer these questions before submitting your issue. Thanks!
### What version of Go are you using (`go version`)?
v 1.11
### Does this issue reproduce with the latest release?
yes
### What operating system and processor architecture are you using (`go env`)?
```
GOARCH="amd64"
GOBIN="/Users/adamtcarter/go/bin"
GOCACHE="/Users/adamtcarter/Library/Caches/go-build"
GOEXE=""
GOFLAGS=""
GOHOSTARCH="amd64"
GOHOSTOS="darwin"
GOOS="darwin"
GOPATH="/Users/adamtcarter/go"
GOPROXY=""
GORACE=""
GOROOT="/usr/local/go"
GOTMPDIR=""
GOTOOLDIR="/usr/local/go/pkg/tool/darwin_amd64"
GCCGO="gccgo"
CC="clang"
CXX="clang++"
CGO_ENABLED="1"
GOMOD=""
CGO_CFLAGS="-g -O2"
CGO_CPPFLAGS=""
CGO_CXXFLAGS="-g -O2"
CGO_FFLAGS="-g -O2"
CGO_LDFLAGS="-g -O2"
PKG_CONFIG="pkg-config"
GOGCCFLAGS="-fPIC -m64 -pthread -fno-caret-diagnostics -Qunused-arguments -fmessage-length=0 -fdebug-prefix-map=/var/folders/7h/f4ryg4z10xd13dy_qf221grc0000gn/T/go-build718587663=/tmp/go-build -gno-record-gcc-switches -fno-common"
```
### What did you do?
Use web sockets in go while targeting js,wasm
### What did you expect to see?
NA
### What did you see instead?
NA
If it makes sense, please add a WebSocket client in x/net/websocket when targeting js,wasm. I was digging around some of the code and it looks like it would be possible by taking a similar approach to what was taken with http.Request calling the native browser `fetch` api. Let me know if it's viable and I can take a stab at it. | NeedsInvestigation,FeatureRequest | low | Critical |
354,797,784 | pytorch | Cannot run torch in different sub-interpreters | ## Issue description
It seems that torch cannot be imported in two different sub-interpreters, as they are described in https://docs.python.org/2/c-api/init.html#sub-interpreter-support.
## Code example
#include <Python.h>
int main(int argc, char **argv) {
Py_Initialize();
PyEval_InitThreads();
PyThreadState *thread1 = Py_NewInterpreter();
PyThreadState *thread2 = Py_NewInterpreter();
PyEval_ReleaseLock();
PyEval_AcquireLock();
PyThreadState_Swap(thread1);
PyRun_SimpleString("import torch");
PyEval_ReleaseLock();
PyEval_AcquireLock();
PyThreadState_Swap(thread2);
PyRun_SimpleString("import torch"); // This is where the error occurs.
PyEval_ReleaseLock();
Py_Finalize();
return 0;
}
## Result
Traceback (most recent call last):
File "<string>", line 1, in <module>
File "/usr/data/bdawagne/python/lib/python3.6/site-packages/torch/__init__.py", line 186, in <module>
from .tensor import Tensor
File "/usr/data/bdawagne/python/lib/python3.6/site-packages/torch/tensor.py", line 12, in <module>
class Tensor(torch._C._TensorBase):
File "/usr/data/bdawagne/python/lib/python3.6/site-packages/torch/tensor.py", line 172, in Tensor
""")
RuntimeError: method 'detach' already has a docstring
## System Info
PyTorch version: 0.4.1
Is debug build: No
CUDA used to build PyTorch: 9.0.176
OS: Fedora 28 (Twenty Eight)
GCC version: (GCC) 8.1.1 20180712 (Red Hat 8.1.1-5)
CMake version: version 3.11.2
Python version: 3.6
Is CUDA available: Yes
CUDA runtime version: 9.1.85
GPU models and configuration:
GPU 0: GeForce GTX 1080 Ti
GPU 1: GeForce GTX 1080 Ti
Nvidia driver version: 396.45
cuDNN version: Could not collect
Versions of relevant libraries:
[pip] Could not collect
[conda] Could not collect
cc @yf225 @glaringlee | todo,needs reproduction,module: cpp,triaged | low | Critical |
354,816,856 | pytorch | Better dev docs for writing native CPU kernels with Vec256 | There have been multiple PRs that put non-arch-specific CPU kernels in `native/cpu`. We need to
1. Rename `native/cpu`
2. Have better dev docs on how to use `Vec256`.
cc @colesbury @cpuhrsch | triaged,module: vectorization | low | Minor |
354,836,920 | rust | Add filter to `rustc_on_unimplemented` for `self_has_method="foo"` | Add a way to query on specific methods and traits being available to `rustc_on_unimplemented` for more targeted suggestions. | C-enhancement,A-diagnostics,T-compiler,F-on_unimplemented | low | Minor |
354,884,007 | flutter | Facilitate Proguard on Android | Facilitate Proguard on Android.
| platform-android,tool,customer: dream (g3),P3,team-android,triaged-android | low | Minor |
354,897,358 | rust | Can't compare reference to ptr but reverse works | ```rust
fn main() {
let a = 1;
let b = &mut a as *mut _;
// Ok:
b == &mut a;
// Not Ok:
&mut a == b;
}
```
Surprisingly, the second comparison errors with:
```shell
error[E0277]: the trait bound `&mut {integer}: std::cmp::PartialEq<*mut {integer}>` is not satisfied
--> src/main.rs:6:12
|
6 | &mut a == b;
| ^^ can't compare `&mut {integer}` with `*mut {integer}`
|
= help: the trait `std::cmp::PartialEq<*mut {integer}>` is not implemented for `&mut {integer}`
```
I believe reference vs ptr comparisons should be legal as the reverse is legal - and if the error message is to be believed, it's just as simple as adding a PartialEq impl for raw ptrs? | T-lang,C-bug | low | Critical |
354,920,577 | pytorch | [feature request] padding for torch.cat | Currently, `torch.cat` only allows to concatenate equal-dimensional Tensors (except in the dimension catting).
For example:
```python
>>> x = torch.ones(2, 3)
>>> y = torch.zeros(1, 3)
>>> torch.cat([x, y], dim=0)
tensor([[1., 1., 1.],
[1., 1., 1.],
[0., 0., 0.]])
>>> x = torch.ones(2, 3)
>>> y = torch.zeros(1, 4)
>>> torch.cat([x, y], dim=0)
RuntimeError: invalid argument 0: Sizes of tensors must match except in dimension 0. Got 3 and 4 in dimension 1 at ../aten/src/TH/generic/THTensorMoreMath.cpp:1348
```
The proposal is to add a `padding` option to `torch.cat`, which will find the maximum size of Tensors in each dimension, and will place each Tensor within that larger padded Tensor.
```python
torch.cat(TensorList, dim, pad=True, pad_value=0.0)
```
Example:
```python
>>> x = torch.ones(2, 3)
>>> y = torch.zeros(1, 4)
>>> torch.cat([x, y], dim=0, pad=True, pad_value=0.5)
tensor([[1., 1., 1., 0.5],
[1., 1., 1., 0.5],
[0., 0., 0., 0.]])
```
If the TensorList has size mismatches in multiple dimensions, the output size will be computed by finding the max of sizes in each dimension, except the dimension being concatenated.
For example:
```python
>>> x = torch.ones(1, 2, 3, 4, 5)
>>> y = torch.ones(3, 9, 2, 5, 1)
>>> torch.cat([x, y], dim=0, pad=True).size()
torch.Size([4, 9, 3, 5, 5])
``` | triaged,enhancement,module: viewing and reshaping | medium | Critical |
354,940,021 | godot | A D-Pad button presses two buttons for Gamecube controllers / joysticks and Mayflash adaptors | **Godot version:**
3.0.6
**OS/device including version:**
Mac OS X El Capitan
Version 10.11.6 (15G20015)
**Issue description:**
When I use a Nintendo Gamecube controller that’s connected to a USB Mayflash adaptor, pressing only one d-pad button at a time results in multiple buttons being pressed at the same time.
Here’s what the Output says when I press each button and print out which button was pressed:
Pressing left on the d-pad:
Output prints: Controller pressed buttons #15,#14,#15 and #14.
I was expecting it to only say one of these numbers, and only once per button press.
Pressing down: Output prints: #14,13,14 and 13
Same expectation.
Pressing Right: Prints 13, 13, 15 and 15.
Pressing up: Prints 12 and 12.
I don’t think it’s because I pressed the button for too long because I can press any other button only once with ease.
Tested with two different Gamecube controllers,
one of them is 1st party from Nintendo,
the other is a third party “SUPERPAD” from InterAct.
Both controller D-pads work fine when tested with Joystick and Gamepad Tester:
https://download.freedownloadmanager.org/Mac-OS/Joystick-And-Gamepad-Tester/FREE-7.1.html
**Steps to reproduce:**
Plug in a Gamecube controller into a USB Mayflash adaptor.
Plug the adaptor into an iMac (Intel).
Run attached Godot Project.
Run the scene.
Press D-pad buttons one at a time and read the Output messages provided by the autoloaded script.
You can also test any other USB joystick.
Note: the script is autoloaded. It automatically detects which gamecube controller is pushing buttons. Without it, button testing might not work because: simply using Input.is_joy_button_pressed(0, 12) might not work since the active gamecube controller # is always random each time you run the scene.
Please fix. Thank you!
**Minimal reproduction project:**
See attached zip file.
[GamecubeControllerTest.zip](https://github.com/godotengine/godot/files/2330143/GamecubeControllerTest.zip)
If the project doesn't work, please make a new one with a scene and set the project to autoload this script:
[buttonTest.gd.zip](https://github.com/godotengine/godot/files/2330145/buttonTest.gd.zip)
| bug,confirmed,topic:thirdparty,topic:input | medium | Major |
354,976,230 | pytorch | [caffe2] Implement Gather for any value of `axis` | Fix this: https://github.com/pytorch/pytorch/blob/master/caffe2/onnx/backend.cc#L629 which is because the implementation of Gather does not support axes other than 1 | caffe2 | low | Minor |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.