id
int64 393k
2.82B
| repo
stringclasses 68
values | title
stringlengths 1
936
| body
stringlengths 0
256k
⌀ | labels
stringlengths 2
508
| priority
stringclasses 3
values | severity
stringclasses 3
values |
---|---|---|---|---|---|---|
304,080,322 | You-Dont-Know-JS | Async & Performance Chapter 4 for..of completion signal to iterator | The section is called "Stopping the generator" and here it is:
> In the previous example, it would appear the iterator instance for the *something() generator was basically left in a suspended state forever after the break in the loop was called.<br>
> But there's a hidden behavior that takes care of that for you. "Abnormal completion" (i.e., "early termination") of the for..of loop -- generally caused by a break, return, or an uncaught exception -- sends a signal to the generator's iterator for it to terminate.<br>
> Note: Technically, the for..of loop also sends this signal to the iterator at the normal completion of the loop. For a generator, that's essentially a moot operation, as the generator's iterator had to complete first so the for..of loop completed. However, custom iterators might desire to receive this additional signal from for..of loop consumers.<br>
> While a for..of loop will automatically send this signal, you may wish to send the signal manually to an iterator; you do this by calling return(..).
The third paragraph is what I have problems comprehending, how does the `for..of` loop send the completion signal to the iterator it is iterating? I would assume by calling `return`, but this is certainly not true because the return method in the following snippet is never called (`return` would not have been called even if we used a generator):
```
const x = {
[Symbol.iterator]: function() {
return this;
},
next: function() {
typeof y !== 'undefined' ? y++ : y = 0;
return {
value: y,
done: y > 10,
};
},
return: function() {
console.log('"return" was called!');
return {done: true};
}
};
for (let a of x) {
// this is insignificant
console.log(a + 10 * 2 / 3);
}
// If the 'for..of' loop sends a completion signal by calling 'return' after all,
// 'return was called' should have been printed to the console by this point.
```
So I am assuming that you are referring to the `finally` block inside of a generator, which is always reached ? But then that is certainly not a moot operation (as stated in the paragraph I quoted). | for second edition | medium | Major |
304,081,442 | TypeScript | In JS, module.exports should support property assignments as declaration | ```ts
// @noEmit: true
// @allowJs: true
// @checkJs: true
// @strict: true
// @Filename: a.js
// from debug
var debug = module.exports = create.debug = create['default'] = create;
function create() {
function d() {
var curr = +new Date()
d.curr = curr
}
return d
}
function assert() {
}
debug.assert = assert
```
**Expected behavior:**
No errors, and the `debug` module should have exports `{ debug, default, assert }` and also be callable.
**Actual behavior:**
Lots of different errors, and no exported members. Moving the function `create` before the exports assignments adds the export `debug`, but that's it. | Bug,checkJs,Domain: JavaScript | low | Critical |
304,082,498 | TypeScript | In JS, module.exports should support "defaulted assignment" declarations | Javascript allows you to write `var a = global.a || {}` in order to use a previously declared `a` if present. This should be allowed in Javascript `module.exports` assignments too.
```ts
// @noEmit: true
// @allowJs: true
// @checkJs: true
// @Filename: a.js
// from url-search-params
function A() {
}
module.exports = global.A || A
```
**Expected behavior:**
No error, and url-search-params is a callable module.
**Actual behavior:**
Lots of errors, and no module. | Bug,checkJs,Domain: JavaScript | low | Critical |
304,090,307 | rust | Tracking issue for future-incompatibility lint `unstable_name_collisions` | This is the **summary issue** for the `unstable_name_collisions` future-incompatibility lint and other related errors. The goal of this page is describe why this change was made and how you can fix code that is affected by it. It also provides a place to ask questions or register a complaint if you feel the change should not be made. For more information on the policy around future-compatibility warnings, see our [breaking change policy guidelines][guidelines].
[guidelines]: https://github.com/rust-lang/rfcs/blob/master/text/1122-language-semver.md
### What is the warning for?
Rust's standard library is evolving, and will sometimes add new functions we found useful, e.g. `Ord::clamp` or `Iterator::flatten`. Unfortunately, there will often be popular third-party packages already implemented the same function with the same name. The compiler, when seeing the name `flatten`, cannot decide whether you want the one from the standard library or the third-party library, and gives up with the [multiple applicable items in scope](https://doc.rust-lang.org/error-index.html#E0034) error.
As an inference breakage canary, the compiler will emit a warning whenever it detected a name conflict with an unstable standard library function.
```rust
extern crate itertools;
use itertools::Itertools;
fn main() {
println!(
"{:?}",
[[1, 2], [3, 4]].iter().flatten().collect::<Vec<_>>()
//~^ WARN a method with this name will be added to the standard library in the future
);
}
```
To stop this warning and the future hard error, you could use fully-qualified name to explicitly tell the compiler to use the third-party function:
```rust
extern crate itertools;
use itertools::Itertools;
fn main() {
println!(
"{:?}",
Itertools::flatten([[1, 2], [3, 4]].iter()).collect::<Vec<_>>()
// ^~~~~~~~~~~~~~~~~~~.......................~
// explicitly use the `flatten` method from the `Itertools` trait.
);
}
```
### When will this warning become a hard error?
This warning will be emitted for every unstable library feature having name conflicts. When a particular library feature is stabilized, this name conflict will cause a hard error.
Please follow the tracking issue for each library feature for their stabilization progress. Some current unstable method which is known to cause conflict in the wild are:
- [ ] `Vec::try_reserve` — #48043
- [ ] `Ord::clamp` — #44095 | A-lints,T-compiler,C-future-incompatibility,C-tracking-issue | medium | Critical |
304,102,943 | go | cmd/go: file positions reported by 'go test' differ for compile errors and vet errors | Given a single file with a trivial syntax error in the current directory:
```
$ cat f.go
package p
func
$ go build f.go
# command-line-arguments
./f.go:4:1: syntax error: unexpected EOF, expecting name or (
$ gofmt f.go
f.go:3:6: expected 'IDENT', found 'EOF'
```
The compiler likes to use the `./` prefix, while the older packages like `go/parser` don't. Is there a good reason for this inconsistency? If not, I imagine that they should be consistent.
Labelling as NeedsDecision as it's not immediately clear to me if there should be a fix, and what that fix would look like.
/cc @griesemer @mdempsky | NeedsFix | low | Critical |
304,102,966 | flutter | Add support for printing to logcat at different levels | Internal: b/324516014
## Steps to Reproduce
Generate logging entries with `logging: "^0.11.3+1"` for any Flutter log level
```dart
import 'package:logging/logging.dart';
final Logger log = new Logger('Example');
Logger.root.level = Level.ALL;
Logger.root.onRecord.listen((LogRecord rec) {
print('${rec.level.name}: ${rec.message}');
});
log.warning("Warning!");
log.severe("Severe error!");
```
## Logs
logcat output:
```
03-10 15:36:36.565: I/flutter(29953): WARNING: Warning!
03-10 15:36:36.566: I/flutter(29953): SEVERE: Severe error!
```
Android ADB log will show logging at native level of INFO for any Flutter log level.
## Flutter Doctor
Paste the output of running `flutter doctor -v` here.
```
[√] Flutter (Channel master, v0.1.9-pre.14, on Microsoft Windows [Version 10.0.16299.248], locale en-US)
• Flutter version 0.1.9-pre.14 at E:\flutter
• Framework revision eaa9848fd4 (10 days ago), 2018-02-28 17:53:38 -0800
• Engine revision 6607231638
• Dart version 2.0.0-dev.31.0.flutter-1aaebedd70
[√] Android toolchain - develop for Android devices (Android SDK 27.0.3)
• Android SDK at C:\Users\jens\AppData\Local\Android\sdk
• Android NDK location not configured (optional; useful for native profiling support)
• Platform android-27, build-tools 27.0.3
• Java binary at: E:\Program Files\Android\Android Studio\jre\bin\java
• Java version OpenJDK Runtime Environment (build 1.8.0_152-release-915-b01)
• All Android licenses accepted.
[√] Android Studio (version 3.0)
• Android Studio at E:\Program Files\Android\Android Studio
• Java version OpenJDK Runtime Environment (build 1.8.0_152-release-915-b01)
[√] VS Code (version 1.21.0)
• VS Code at C:\Program Files\Microsoft VS Code
• Dart Code extension version 2.10.0
[√] Connected devices (1 available)
• LG H910 • LGH9107d4f80b5 • android-arm64 • Android 7.0 (API 24)
• No issues found!
``` | c: new feature,framework,engine,P2,customer: huggsy (g3),team-engine,triaged-engine | low | Critical |
304,108,803 | TypeScript | getDefinitionAtPosition doesn't distinguish different kinds in a merged declaration | **TypeScript Version:** 2.7.2
**Search Terms:** getDefinitionAtPosition kind
**Code**
```ts
namespace A { export interface B {} }
class A {}
interface A {}
var x: A; // do getDefinitionAtPosition on the A reference here
```
**Expected behavior:**
In the result, each of the individual declarations should have the appropriate `kind`:
```js
[
{ fileName: 'test.ts', textSpan: { start: 10, length: 1 }, kind: 'module', name: 'A', ... },
{ fileName: 'test.ts', textSpan: { start: 44, length: 1 }, kind: 'class', name: 'A', ... },
{ fileName: 'test.ts', textSpan: { start: 59, length: 1 }, kind: 'interface', name: 'A', ... }
]
```
**Actual behavior:**
All declarations have the same `kind`:
```js
[
{ fileName: 'test.ts', textSpan: { start: 10, length: 1 }, kind: 'class', name: 'A', ... },
{ fileName: 'test.ts', textSpan: { start: 44, length: 1 }, kind: 'class', name: 'A', ... },
{ fileName: 'test.ts', textSpan: { start: 59, length: 1 }, kind: 'class', name: 'A', ... }
]
``` | Bug,Help Wanted,Good First Issue,PursuitFellowship | low | Major |
304,146,221 | material-ui | [Chip] Add "selected" prop | <!--- Provide a general summary of the issue in the Title above -->
<!--
Thank you very much for contributing to Material-UI by creating an issue! ❤️
To avoid duplicate issues we ask you to check off the following list.
-->
<!-- Checked checkbox should look like this: [x] -->
- [x] I have searched the [issues](https://github.com/mui-org/material-ui/issues) of this repository and believe that this is not a duplicate.
## Expected Behavior
<!---
If you're describing a bug, tell us what should happen.
If you're suggesting a change/improvement, tell us how it should work.
-->
`Chip` should have an `active` prop to set the `Chip` to be visually active or not.
## Current Behavior
<!---
If describing a bug, tell us what happens instead of the expected behavior.
If suggesting a change/improvement, explain the difference from current behavior.
-->
Currently, the only way to make a `Chip` active is to click on it with `onClick` set.
## Steps to Reproduce (for bugs)
<!---
Provide a link to a live example (you can use codesandbox.io) and an unambiguous set of steps to reproduce this bug.
Include code to reproduce, if relevant (which it most likely is).
This codesandbox.io template _may_ be a good starting point:
https://codesandbox.io/s/github/mui-org/material-ui/tree/v1-beta/examples/create-react-app
If YOU DO NOT take time to provide a codesandbox.io reproduction, should the COMMUNITY take time to help you?
-->
## Context
<!---
How has this issue affected you? What are you trying to accomplish?
Providing context helps us come up with a solution that is most useful in the real world.
-->
## Your Environment
<!--- Include as many relevant details about the environment with which you experienced the bug. -->
| Tech | Version |
|--------------|---------|
| Material-UI | beta.36 |
| React | 16.2.0 |
| browser | |
| etc | |
| new feature,design: material,component: chip | low | Critical |
304,162,075 | gin | Proposal of a new implementation method of LoadHTMLGlob | I'm very grateful that I usually use **gin** to create web services.
However, I felt that LoadHTMLGlob is a slightly bad implementation.
When loading a nested HTML file with LoadHTMLGlob, you must define it in the tmpl file.
If you implement the following implementation, how do you think HTML can be called immediately?
*gin*
```go
package gin
import (
"fmt"
"html/template"
"net/http"
"os"
"path/filepath"
"strings"
)
var filepathArray []string
func LoadHTMLGlob(path string) {
var rootDir string
if strings.HasSuffix(path, "*") {
rootDir = strings.Split(path, "/")[0]
}
err := filepath.Walk(rootDir, func(p string, info os.FileInfo, err error) error {
if strings.HasSuffix(p, ".html") {
filepathArray = append(filepathArray, p)
}
return nil
})
if err != nil {
panic(err)
}
}
func GET(endpoint, file string) {
var path string
for _, p := range filepathArray {
if strings.HasSuffix(p, file) {
path = p
}
}
fmt.Println(endpoint + "->" + path)
http.HandleFunc(endpoint, func(w http.ResponseWriter, r *http.Request) {
tmpl, err := template.ParseFiles(path)
if err != nil {
panic(err)
}
err = tmpl.Execute(w, nil)
if err != nil {
panic(err)
}
})
}
func Run(port string) {
http.ListenAndServe(port, nil)
}
```
User write main.go
```go
package main
import (
"github.com/konojunya/html-glob-handler/gin"
)
func main() {
gin.LoadHTMLGlob("views/*")
gin.GET("/", "home/index.html")
gin.Run(":8000")
}
```
What I'd like to say is not to make gin.Run possible, but I also want LoadHTMLGlob to be able to read HTML files in deep Directory.
Thanks.
| feature | low | Critical |
304,187,383 | godot | C# auto complete for _Input spits out an invalid variable name '@event' | **Godot version:**
3.0.2+
**OS/device including version:**
Windows 10
**Issue description:**
When using windows 10 and vs code and you try to autocomplete the `_Input` method it spits out
```csharp
public override void _Input(InputEvent @event)
{
}
```
**Steps to reproduce:**
Just attempt to auto complete the input field
**Suggestion:**
1. Change the variable name to `input` since ~~`@event` is invalid and~~ `event` is a reserved C# keyword. | enhancement,usability,topic:dotnet | low | Major |
304,196,418 | scrcpy | Show touch | In developer options, there is an option "show taps", that whenever you touch the screen, a white circle appears.
For some reason, it doesn't appear when using this app.
Please let us have it.
It can be useful for screenshots and screen-video capture. | feature request,showtaps | medium | Critical |
304,198,122 | go | go: add more examples to go/* package documentation | It can be hard to figure out how to use the go/* packages. More examples will help.
Coming up with useful, short examples that demonstrate these packages well will be hard and ongoing and probably require some discussion, hence this meta-issue.
A complimentary wiki page (what would a good name for this page be?), in the vein of https://github.com/golang/go/wiki/cgo , would be helpful for larger or more complicated examples, some notes about when to use which package, links to helpful packages in golang.org/x/tools/go, and for various tips and tricks, but having playable examples of the basics will be very helpful.
Related issue: #23603 so that examples can rely on go/parser and go/build can still be playable even if they hit the fs. I think this should be okay with the following rules:
1. self contained example should be preferred.
2. the example can only parse files in its own package (to avoid unrelated changes breaking them)
3. the files accessed in the example must be included regardless of build tag (so that they're always easily reachable from the "Package files" section in godoc)
4. the example should rely only on features under the Go 1 compatibility agreement (to avoid having to be updated when the package itself is changed)
Here are some potential examples to include:
An example in go/parser that uses go/build and ParseDir to get the *ast.Package given an import path. (This seems to be a common stumbling block, in my experience).
Some examples in go/constant using BinaryOp and Compare and some of the conversions/constructors.
An example in go/token of using the FileSet to turn a Pos into a Position.
Based on s/owners, cc: @griesemer @alandonovan @rsc @robpike | Documentation,help wanted,NeedsInvestigation | low | Major |
304,200,823 | scrcpy | Add auto-update to the app | As the title says | feature request | low | Major |
304,200,903 | three.js | CameraHelper: .clone() is broken | ##### Description of the problem
`CameraHelper::constructor` expects a `Camera` argument, but since `CameraHelper::clone` is not overwritten it will forward to `LineSegments::constructor` which expects a geometry and a material.
As a result `cameraHelper.camera` won't be set and `cameraHelper.clone()` throws via `.update()` (see https://jsfiddle.net/bbnk59af/).
Overwriting `CameraHelper::clone` to account for its own constructor solves the issue (see https://jsfiddle.net/bbnk59af/1/):
```
THREE.CameraHelper.prototype.clone = function () {
var clone = new this.constructor(this.camera).copy(this);
return clone;
}
```
I am not sure what the intended behaviour is in the case that `cameraHelper.clone()` gets called as a child of `scene.clone()`. The cameras and their helpers would be cloned individually, but the cloned helpers would point to the original cameras instead of the cloned ones.
##### Three.js version
- [X] Dev
- [X] r90
##### Browser
- [x] All of them
##### OS
- [x] All of them | Enhancement | low | Critical |
304,205,906 | godot | Next pass in CanvasItemMaterial seems to do nothing? | <!-- Please search existing issues for potential duplicates before filing yours:
https://github.com/godotengine/godot/issues?q=is%3Aissue
-->
**Godot version:**
eceba5a
**OS/device including version:**
OS: Ubuntu Linux 17.10 64b.
OpenGL version string: 4.6.0 NVIDIA 387.34
OpenGL shading language version string: 4.60 NVIDIA
Video device: GeForce GT 630/PCIe/SSE2
**Issue description:**
I am not entirely sure if this is human error, since the documentation is sparse, but setting a ShaderMaterial as next_step of a CanvasItemMaterial seems to have no effect. I included a little test displaying the shader working when set as material directly, and a "_wrong" version where it's set as the next step.
**Steps to reproduce:**
Set a shader material as the next_step of a CanvasItemMaterial.
**Minimal reproduction project:**
[b.zip](https://github.com/godotengine/godot/files/1800991/b.zip)
| enhancement,topic:rendering,confirmed | medium | Critical |
304,211,655 | rust | `Span` issues with `FromStr` in unstable `proc_macro` | While writing improved tests for `proc_macro2`, I noticed some surprising behaviour of the `FromStr` impl for `proc_macro::TokenStream`.
When parsing a string with `FromStr`, a token parsed from the very beginning of the string can have a different `source_file()` than a token parsed elsewhere. For example:
```rust
let tokens = "aaa\nbbb".parse::<proc_macro::TokenStream>().unwrap().into_iter().collect::<Vec<_>>();
println!("span: {:?}", tokens[0].span);
println!("source_file: {:?}", tokens[0].span.source_file());
println!("");
println!("span: {:?}", tokens[1].span);
println!("source_file: {:?}", tokens[1].span.source_file());
```
Will produce the output:
```
span: Span(Span { lo: BytePos(6734829), hi: BytePos(6734832), ctxt: #0 })
source_file: SourceFile { path: ProcMacroSourceCode, is_real: false }
span: Span(Span { lo: BytePos(50), hi: BytePos(58), ctxt: #25 })
source_file: SourceFile { path: Real("pm_test.rs"), is_real: true }
```
I would've expected both tokens to have the same context, and similar BytePos-es. Specifically I would have expected both of these tokens to have a span located in a fake ProcMacroSourceCode file, but set to resolve in the context of the enclosing scope (as if span.resolved_at(Span::call_site()) was called). | E-needs-test,T-compiler,A-macros-2.0,C-bug | low | Minor |
304,236,161 | youtube-dl | [raywenderlich] Add support for authentication | ## Please follow the guide below
- You will be asked some questions and requested to provide some information, please read them **carefully** and answer honestly
- Put an `x` into all the boxes [ ] relevant to your *issue* (like this: `[x]`)
- Use the *Preview* tab to see what your issue will actually look like
---
### Make sure you are using the *latest* version: run `youtube-dl --version` and ensure your version is *2018.03.10*. If it's not, read [this FAQ entry](https://github.com/rg3/youtube-dl/blob/master/README.md#how-do-i-update-youtube-dl) and update. Issues with outdated version will be rejected.
- [x] I've **verified** and **I assure** that I'm running youtube-dl **2018.03.10**
### Before submitting an *issue* make sure you have:
- [x] At least skimmed through the [README](https://github.com/rg3/youtube-dl/blob/master/README.md), **most notably** the [FAQ](https://github.com/rg3/youtube-dl#faq) and [BUGS](https://github.com/rg3/youtube-dl#bugs) sections
- [x] [Searched](https://github.com/rg3/youtube-dl/search?type=Issues) the bugtracker for similar issues including closed ones
- [x] Checked that provided video/audio/playlist URLs (if any) are alive and playable in a browser
### What is the purpose of your *issue*?
- [x] Bug report (encountered problems with youtube-dl)
- [ ] Site support request (request for adding support for a new site)
- [ ] Feature request (request for a new functionality)
- [ ] Question
- [ ] Other
---
### The following sections concretize particular purposed issues, you can erase any section (the contents between triple ---) not applicable to your *issue*
---
### If the purpose of this *issue* is a *bug report*, *site support request* or you are not completely sure provide the full verbose output as follows:
Add the `-v` flag to **your command line** you run youtube-dl with (`youtube-dl -v <your command line>`), copy the **whole** output and insert it here. It should look similar to one below (replace it with **your** log inserted between triple ```):
```
st0g1e$ youtube-dl -v -u "[email protected]" -p fakepassword https://videos.raywenderlich.com/courses/115-server-side-swift-with-vapor/lessons/1
[debug] System config: []
[debug] User config: []
[debug] Custom config: []
[debug] Command-line args: [u'-v', u'-u', u'PRIVATE', u'-p', u'PRIVATE', u'https://videos.raywenderlich.com/courses/115-server-side-swift-with-vapor/lessons/1']
[debug] Encodings: locale UTF-8, fs utf-8, out UTF-8, pref UTF-8
[debug] youtube-dl version 2018.03.10
[debug] Python version 2.7.10 (CPython) - Darwin-17.4.0-x86_64-i386-64bit
[debug] exe versions: ffmpeg 3.4.2-tessus
[debug] Proxy map: {}
[RayWenderlich] 115-server-side-swift-with-vapor/1: Downloading webpage
[RayWenderlich] Downloading playlist 115-server-side-swift-with-vapor - add --no-playlist to just download video
[download] Downloading playlist: Server Side Swift with Vapor
[RayWenderlich] playlist Server Side Swift with Vapor: Collected 30 video ids (downloading 30 of them)
[download] Downloading video 1 of 30
[RayWenderlich] 115-server-side-swift-with-vapor/1: Downloading webpage
[vimeo] Downloading login page
[vimeo] Logging in
ERROR: Unable to log in: bad username or password
Traceback (most recent call last):
File "/usr/local/bin/youtube-dl/youtube_dl/extractor/vimeo.py", line 60, in _login
'Referer': self._LOGIN_URL,
File "/usr/local/bin/youtube-dl/youtube_dl/extractor/common.py", line 634, in _download_webpage
res = self._download_webpage_handle(url_or_request, video_id, note, errnote, fatal, encoding=encoding, data=data, headers=headers, query=query)
File "/usr/local/bin/youtube-dl/youtube_dl/extractor/common.py", line 539, in _download_webpage_handle
urlh = self._request_webpage(url_or_request, video_id, note, errnote, fatal, data=data, headers=headers, query=query)
File "/usr/local/bin/youtube-dl/youtube_dl/extractor/common.py", line 528, in _request_webpage
raise ExtractorError(errmsg, sys.exc_info()[2], cause=err)
ExtractorError: Unable to download webpage: HTTP Error 418: I'm a teapot (caused by HTTPError()); please report this issue on https://yt-dl.org/bug . Make sure you are using the latest version; type youtube-dl -U to update. Be sure to call youtube-dl with the --verbose flag and include its complete output.
Traceback (most recent call last):
File "/usr/local/bin/youtube-dl/youtube_dl/YoutubeDL.py", line 785, in extract_info
ie_result = ie.extract(url)
File "/usr/local/bin/youtube-dl/youtube_dl/extractor/common.py", line 439, in extract
self.initialize()
File "/usr/local/bin/youtube-dl/youtube_dl/extractor/common.py", line 397, in initialize
self._real_initialize()
File "/usr/local/bin/youtube-dl/youtube_dl/extractor/vimeo.py", line 457, in _real_initialize
self._login()
File "/usr/local/bin/youtube-dl/youtube_dl/extractor/vimeo.py", line 66, in _login
expected=True)
ExtractorError: Unable to log in: bad username or password
```
| account-needed | low | Critical |
304,279,003 | flutter | Show more granular details during gradle build | The `Running 'gradlew assembleDebug'...` takes a long time and shows no indication of what it's currently doing. Would be nice to break it up more like #14715 and show more details. Should be easy since flutter.gradle is already neatly broken up. | platform-android,tool,t: gradle,P3,team-android,triaged-android | low | Critical |
304,293,799 | go | cmd/go: build cache does not check #included headers for changes | go 1.10 linux/amd64
The `go build` and `go test` commands don't rebuild packages if included cgo files changed. I guess a solution would be to run the preprocessor first or disable caching for packages that use cgo altogether. | help wanted,NeedsInvestigation | medium | Critical |
304,302,860 | flutter | Flutter driver test fails when invoked directly via `dart.exe` | **I just started setting up our Flutter App testing added the following dependencies.
in `pubspec.yaml`
```yml
flutter_driver:
sdk:
flutter
```
`test_driver` folder in the project
and add test file with extension `.dart`
and created Flutter-Driver folder for creating scripts.
But unfortunately, I can't run the test. I got the following error**
## while debugging
```
exception = DriverError: Could not determine URL to connect to application.
Either the VM_SERVICE_URL environment variable should be set, or
```
when you run the script
## while running the script
```
C:\Users\KRISHNA_CHIMPLE\Documents\GitHub\git\flutter\bin\cache\dart-sdk\bin\dart.exe --checked --enable-vm-service:65143 C:\Users\KRISHNA_CHIMPLE\Downloads\flutter_app-master\flutter_app-master\test_driver\Navigation_test.dart
Observatory listening on http://127.0.0.1:65143/
00:00 +0: flutter app transitions (setUpAll)
00:00 +0 -1: flutter app transitions (setUpAll) [E]
DriverError: Could not determine URL to connect to application.
Either the VM_SERVICE_URL environment variable should be set, or an explicit
URL should be provided to the FlutterDriver.connect() method.
Original error: null
Original stack trace:
null
package:flutter_driver/src/driver/driver.dart 154:7 FlutterDriver.connect
===== asynchronous gap ===========================
dart:async/future_impl.dart 22 _Completer.completeError
package:flutter_driver/src/driver/driver.dart FlutterDriver.connect
===== asynchronous gap ===========================
dart:async/zone.dart 1047 _CustomZone.registerCallback
dart:async/zone.dart 964 _CustomZone.bindCallbackGuarded
dart:async/schedule_microtask.dart 147 scheduleMicrotask
dart:async/future.dart 198 new Future.microtask
package:flutter_driver/src/driver/driver.dart 150:85 FlutterDriver.connect
test_driver\Navigation_test.dart 12:36 main.<fn>.<fn>
===== asynchronous gap ===========================
dart:async/zone.dart 1047 _CustomZone.registerCallback
dart:async/zone.dart 964 _CustomZone.bindCallbackGuarded
dart:async/schedule_microtask.dart 147 scheduleMicrotask
dart:async/future.dart 198 new Future.microtask
test_driver\Navigation_test.dart 11:23 main.<fn>.<fn>
#
``` | tool,t: flutter driver,P2,team-tool,triaged-tool | low | Critical |
304,374,567 | TypeScript | Incorrect Source Map Generation | **TypeScript Version:** 2.5.2
**Search Terms:** source map
**Code**
This is sadly kind of hard to show.
**example.ts**
```ts
import * as fs from 'fs';
import { message } from './extra';
console.log(message, fs.readFileSync(__FILE__));
```
**extra.ts** (force imports to get mangled by tsc)
```ts
export const message = 'example:';
```
```bash
tsc example.ts --sourceMap
```
(you'll get some errors due to minimal example not having nodejs types, they don't matter)
The resulting files:
**example.js**
```js
"use strict";
exports.__esModule = true;
var fs = require("fs");
var extra_1 = require("./extra");
console.log(extra_1.message, fs.readFileSync(__FILE__));
//# sourceMappingURL=example.js.map
```
**example.js.map**
```json
{"version":3,"file":"example.js","sourceRoot":"","sources":["example.ts"],"names":[],"mappings":";;AAAA,uBAAyB;AACzB,iCAAkC;AAClC,OAAO,CAAC,GAAG,CAAC,eAAO,EAAE,EAAE,CAAC,YAAY,CAAC,QAAQ,CAAC,CAAC,CAAC"}
```
You can use the following tool to visualize the mapping https://sokra.github.io/source-map-visualization/
**Expected behavior:**
The "extra_1.message" should have 3 columns specified in the source map. One for "extra_1", one for "." and another for "message"
**Actual behavior:**
Only the column for "extra_1" is present (likely because it's just translating from the original source map?)
This is a problem because browser/node stack traces will ask for the mapping for said columns. So for example in nodejs you may get in cases the column for "." as the source column, not the column for "extra_1". This is possible to mitigate, but ideally should not be needed to. Any difference of more then 1 position is ambigous on how _bad_ the source map error is.
**Related Issues:**
_Imports are very noisy and get unnecesarily mangled_
Fixing this would indirectly solve almost all cases where the issue matters, since traces are unlikely to occur on the import lines, and the following would remove those cases so translating is 1:1 (in case enhancing the map is too hard)
Take `import { message } from './extra';` from above as an example. It gets converted to `var extra_1 = require("./extra");` and `extra_1.message`. This seems like pointlessly defensive code.
I'm sure this is optimized at runtime, so it's not a perf issue necesarily, though still very noisy. It would be much better to if it was `var message = require("./extra").message`, then usage would stay as `message` as per the original source. (Note: imports also suffer from the source map issue, they are a single column + one terminal unknown "thing"[?], instead of 6-7).
For multi-line imports destruction or multi-line assignment would be better as well.
eg.
```js
var __imports = {};
__imports.extra = require('./extra');
var message = imports.extra;
var title = imports.extra;
```
Since the names `message` and `title` would have already been used in the source they are practically like keywords, there's no risk of unintended shadowing outside of complex structures generated by typescript itself; same can not necessarily be said of things such as "extra_1". More noise at the top is much preferred over noise in the actual code.
_Whitespace should not be removed_
Since files are not minified it would be better if empty lines were maintained.
If there is any concern with size (bytes/s while reading) because of whitespace then actually using tabs instead of spaces would yield greater gain, over not removing newlines (`\n`)
_Names are ignored_
Symbol names should probably included. If not by default at least with a flag.
I can understand them not being included to save space. | Bug | low | Critical |
304,405,763 | TypeScript | In JS, reassignment of a constructor function should work. | graceful-fs allows the user to provide a constructor function for the ReadStream class. It then modifies the function’s prototype (including modifying its base class). If no function is provided, then it provides one. This pattern should be supported as long as the provided function is assignable to the default one.
```ts
// @noEmit: true
// @allowJs: true
// @checkJs: true
// @Filename: a.js
var fs = require('fs')
/** @type {{ a: () => void }} */
var legStreams
// from graceful-fs
function patch(process) {
if (process) {
ReadStream = legStreams.a
}
ReadStream.prototype = Object.create(fs.ReadStream)
ReadStream.prototype.open = fs$open
function ReadStream (path, options) {
if (this instanceof ReadStream) {
return fs.ReadStream.apply(this, arguments), this
}
else {
return ReadStream.apply(Object.create(ReadStream.prototype), arguments)
}
}
function fs$open() {
}
// ReadStream should still be newable too!
function createReadStream(path, options) {
return new ReadStream(path, options)
}
``` | Bug,checkJs,Domain: JavaScript | low | Minor |
304,438,425 | go | syscall: Syscall6 not implemented on Solaris | Please answer these questions before submitting your issue. Thanks!
### What version of Go are you using (`go version`)?
go version go1.10 linux/amd64
### Does this issue reproduce with the latest release?
yes
### What operating system and processor architecture are you using (`go env`)?
GOARCH="amd64"
GOBIN=""
GOCACHE="/home/milann/.cache/go-build"
GOEXE=""
GOHOSTARCH="amd64"
GOHOSTOS="linux"
GOOS="linux"
GOPATH="/home/milann/golang"
GORACE=""
GOROOT="/home/milann/go"
GOTMPDIR=""
GOTOOLDIR="/home/milann/go/pkg/tool/linux_amd64"
GCCGO="gccgo"
CC="gcc"
CXX="g++"
CGO_ENABLED="1"
CGO_CFLAGS="-g -O2"
CGO_CPPFLAGS=""
CGO_CXXFLAGS="-g -O2"
CGO_FFLAGS="-g -O2"
CGO_LDFLAGS="-g -O2"
PKG_CONFIG="pkg-config"
GOGCCFLAGS="-fPIC -m64 -pthread -fmessage-length=0 -fdebug-prefix-map=/tmp/go-build031994401=/tmp/go-build -gno-record-gcc-switches"
### What did you do?
I am trying to call shmget, shmat etc. via syscall. On OpenSolaris there is one code for SHM (52) and then shmget etc. functions are sub calls with codes 0,1,2 etc.
If possible, provide a recipe for reproducing the error.
A complete runnable program is good.
A link on play.golang.org is best.
```go
package main
import (
"runtime"
"syscall"
)
const (
IPC_PRIVATE = 0
IPC_CREAT = 01000
)
func main() {
if runtime.GOOS == "solaris" {
id, _, errno := syscall.Syscall6(52, 3, uintptr(int32(IPC_PRIVATE)), uintptr(int32(65536)), uintptr(int32(IPC_CREAT|0777)), 0, 0)
if int(id) == -1 {
println(errno)
}
} else if runtime.GOOS == "linux" {
// 29 is syscall.SYS_SHMGET on amd64, missing on solaris
id, _, errno := syscall.Syscall(29, uintptr(int32(IPC_PRIVATE)), uintptr(int32(65536)), uintptr(int32(IPC_CREAT|0777)))
if int(id) == -1 {
println(errno)
}
}
}
```
### What did you expect to see?
Program compiles ok with GOOS=solaris.
### What did you see instead?
```
GOOS=solaris go build test.go
# command-line-arguments
syscall.Syscall6: call to external function
main.main: relocation target syscall.Syscall6 not defined
main.main: undefined: "syscall.Syscall6"
```
Is this something you are aware of and is there a workaround maybe? For now I also implemented cgo version but I hope just temporary. You can also test with library I am working on:
`GOOS=solaris go get -tags syscall github.com/gen2brain/shm`
| help wanted,OS-Solaris,NeedsFix | medium | Critical |
304,527,611 | youtube-dl | Can't download from Tou.TV extra ("Unable to extract access token") |
- [X] I've **verified** and **I assure** that I'm running youtube-dl **2018.03.10**
- [X] At least skimmed through the [README](https://github.com/rg3/youtube-dl/blob/master/README.md), **most notably** the [FAQ](https://github.com/rg3/youtube-dl#faq) and [BUGS](https://github.com/rg3/youtube-dl#bugs) sections
- [X] [Searched](https://github.com/rg3/youtube-dl/search?type=Issues) the bugtracker for similar issues including closed ones
- [X] Checked that provided video/audio/playlist URLs (if any) are alive and playable in a browser
### What is the purpose of your *issue*?
- [X] Bug report (encountered problems with youtube-dl)
- [ ] Site support request (request for adding support for a new site)
- [ ] Feature request (request for a new functionality)
- [ ] Question
- [ ] Other
---
### If the purpose of this *issue* is a *bug report*, *site support request* or you are not completely sure provide the full verbose output as follows:
```
youtube-dl --verbose -u username_redacted -p password_redacted https://ici.tou.tv/lacher-prise/S01E01
[debug] System config: []
[debug] User config: []
[debug] Custom config: []
[debug] Command-line args: [u'--verbose', u'-u', u'PRIVATE', u'-p', u'PRIVATE', u'https://ici.tou.tv/lacher-prise/S01E01']
[debug] Encodings: locale UTF-8, fs UTF-8, out UTF-8, pref UTF-8
[debug] youtube-dl version 2018.03.10
[debug] Python version 2.7.12 (CPython) - Linux-4.13.0-36-generic-x86_64-with-LinuxMint-18.3-sylvia
[debug] exe versions: ffmpeg 2.8.11-0ubuntu0.16.04.1, ffprobe 2.8.11-0ubuntu0.16.04.1
[debug] Proxy map: {}
[tou.tv] Downloading homepage
[tou.tv] Downloading login page
[tou.tv] Logging in
[tou.tv] Following Redirection
ERROR: Unable to extract access token; please report this issue on https://yt-dl.org/bug . Make sure you are using the latest version; see https://yt-dl.org/update on how to update. Be sure to call youtube-dl with the --verbose flag and include its complete output.
Traceback (most recent call last):
File "/usr/lib/python2.7/dist-packages/youtube_dl/YoutubeDL.py", line 785, in extract_info
ie_result = ie.extract(url)
File "/usr/lib/python2.7/dist-packages/youtube_dl/extractor/common.py", line 439, in extract
self.initialize()
File "/usr/lib/python2.7/dist-packages/youtube_dl/extractor/common.py", line 397, in initialize
self._real_initialize()
File "/usr/lib/python2.7/dist-packages/youtube_dl/extractor/toutv.py", line 88, in _real_initialize
urlh.geturl(), 'access token')
File "/usr/lib/python2.7/dist-packages/youtube_dl/extractor/common.py", line 796, in _search_regex
raise RegexNotFoundError('Unable to extract %s' % _name)
RegexNotFoundError: Unable to extract access token; please report this issue on https://yt-dl.org/bug . Make sure you are using the latest version; see https://yt-dl.org/update on how to update. Be sure to call youtube-dl with the --verbose flag and include its complete output.
```
---
### Description of your *issue*, suggested solution and other information
Can't seem to be able to download anything from Tou.TV extra, although it works on the regular (i.e. non-premium) streams. Has to do with Acess token, but I'm lost. This error message "Unable to extract access token" shows up on any premium content I have tried to download so far.
I'd be much grateful for any help! Regards,
| account-needed | low | Critical |
304,530,984 | flutter | More strongly caveat usage of debugProfileBuildsEnabled and debugProfilePaintsEnabled | Learned the hard way. Seems like Timeline.start/finishSync calls adds performance cost itself on medium complexity layouts in profile mode.
Without tracing, it costs 578ms to produce 28 frames. 242ms in build and 181ms in paint.
With tracing, it costs 1087ms to produce the ~same 29 frames. 420ms in build and 489ms in paint.
Though most tellingly, if only traces were added partially such as paint but not build, it costs 952ms to produce 27 frames but 245ms in build and 543ms in paint.
We should document these caveats. | team,framework,c: performance,d: api docs,a: debugging,P2,team-framework,triaged-framework | low | Critical |
304,531,093 | vscode | Zen Mode Settings | I request a special property for zen mode
zenMode.fontSize and "zenMode.fontWeight": "bold"
So would be different from
editor property settings | feature-request,workbench-zen | medium | Critical |
304,546,819 | flutter | OEM animations | Is it possible to use native animations? Each phone brand has their own animations (I really like my pixel's) and I'd like to use the animations that come with every phone, it breaks the native feel in my opinion when the animations don't match. | platform-android,framework,a: animation,f: material design,a: fidelity,P3,team-android,triaged-android | low | Minor |
304,559,224 | neovim | avoid hit-enter prompt on SIGSTOP (ctrl-z) | I have a plugin installed that is triggered on save, and I'm not sure via what means, neovim currently triggers a buffwrite on `SIGSTOP` (i.e. ctrl-z in normal mode). I tried to capture any events from the signal handling with the logging, but I didn't come up with anything useful (still attached). That is all, however, incidental to this bug report.
The issue is that this plugin, triggered by the save-on-`SIGSTOP` behavior, used `:echoerr` to display an error message. This blocks neovim from being background'd, and the `SIGSTOP` was effectively ignored until I'd addressed the error messages.
When responding to a signal like `SIGSTOP`, it's important that no prolonged blocking activity be carried out before complying, neovim's current behavior could leave it indefinitely hanged in response to a non-tty-generated `SIGSTOP` event expecting neovim to respond accordingly as neovim waits for user input at the command line.
```
NVIM v0.2.3-788-g1d5eec2c6
Build type: Debug
LuaJIT 2.0.5
Compilation: /usr/local/bin/scc -march=native -msse4a -mavx2 -mfma -msha -Wconversion -DNVIM_MSGPACK_HAS_FLOAT32 -DNVIM_UNIBI_HAS_VAR_FROM -g -Wall -Wextra -pedantic -Wno-unused-parameter -Wstrict-prototypes -std=gnu99 -Wimplicit-fallthrough -Wvla -fstack-protector-strong -fdiagnostics-color=auto -DINCLUDE_GENERATED_DECLARATIONS -I/mnt/d/rand/neovim/build/config -I/mnt/d/rand/neovim/src -I/mnt/d/rand/neovim/.deps/usr/include -I/usr/include -I/mnt/d/rand/neovim/build/src/nvim/auto -I/mnt/d/rand/neovim/build/include
Compiled by mqudsi@Blitzkrieg
Features: +acl +iconv +jemalloc +tui
See ":help feature-compile"
system vimrc file: "$VIM/sysinit.vim"
fall-back for $VIM: "/usr/local/share/nvim"
Run :checkhealth for more info
```
[nvim.log](https://github.com/neovim/neovim/files/1804744/nvim.log)
| tui,system | low | Critical |
304,575,032 | flutter | Render as many lines as space allows before ellipsing | I have a fixed sized container with two Text widgets in a column. The first text should take up as much space as needed to render it fully and the second text should render as many lines as the remaining space allows before getting ellipsed.
```dart
new Container(
padding: new EdgeInsets.all(15.0),
width: 150.0,
height: 110.0,
color: Colors.red,
child: new Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
new Text(
'I take up as much space as possible.',
style: const TextStyle(fontWeight: FontWeight.bold),
),
child: new Text(
'The space I have is very limited. I get ellipsed.',
overflow: TextOverflow.ellipsis,
),
],
),
),
```
The second text always gets ellipsed after one line even when there is space to render more lines. There is no way (short of knowing beforehand how many lines the second text can render) to have the text render as many lines as possible before ellipsing. | c: new feature,framework,engine,a: typography,P2,team-engine,triaged-engine | low | Critical |
304,576,008 | flutter | Document the performance impact of Material widget | Point out that likely only one Material instance per elevation is needed to provide the splash for all of its descendent ink wells. Use DecoratedBox if using just for background colors. | framework,f: material design,d: api docs,P2,team-design,triaged-design | low | Major |
304,611,600 | vue | [SSR] 服务端渲染能否增加自定义 TemplateRenderer 或者 提供部分inject的参数? | ### What problem does this feature solve?
当我使用 inject: true 的参数渲染 ssr 页面时,我只想对 renderScripts 这部分做自定义修改,其他继续沿用默认templateRenderer的render**。
如果 我设置了 inject: false,那我必须在 html模板中 添加 各种 {{ render** }} 。
请问有没有更方便的方法?
### What does the proposed API look like?
```
createBundleRenderer({
inject:true,
renderScripts: function() { ... },
})
```
or
```
createBundleRenderer({
inject:true,
templateRenderer: new otherTemplateRenderer(),
})
```
<!-- generated by vue-issues. DO NOT REMOVE --> | feature request | medium | Minor |
304,613,131 | scrcpy | Weird scaling on macOS in Parallels VM | I'm not sure if it's an issue in scrcpy.
With scaling setting "Best for Retina Display" scrcpy running in VM shows cropped image:
<img width="1920" alt="screen shot 2018-03-13 at 06 06 31" src="https://user-images.githubusercontent.com/11808223/37320496-004ac424-2685-11e8-9d34-0661755f2a8c.png">
However, with both "Scaled" and "More Space" it works fine:
<img width="1920" alt="screen shot 2018-03-13 at 06 06 47" src="https://user-images.githubusercontent.com/11808223/37320518-1a2ef842-2685-11e8-9db6-b11affbbcee9.png">
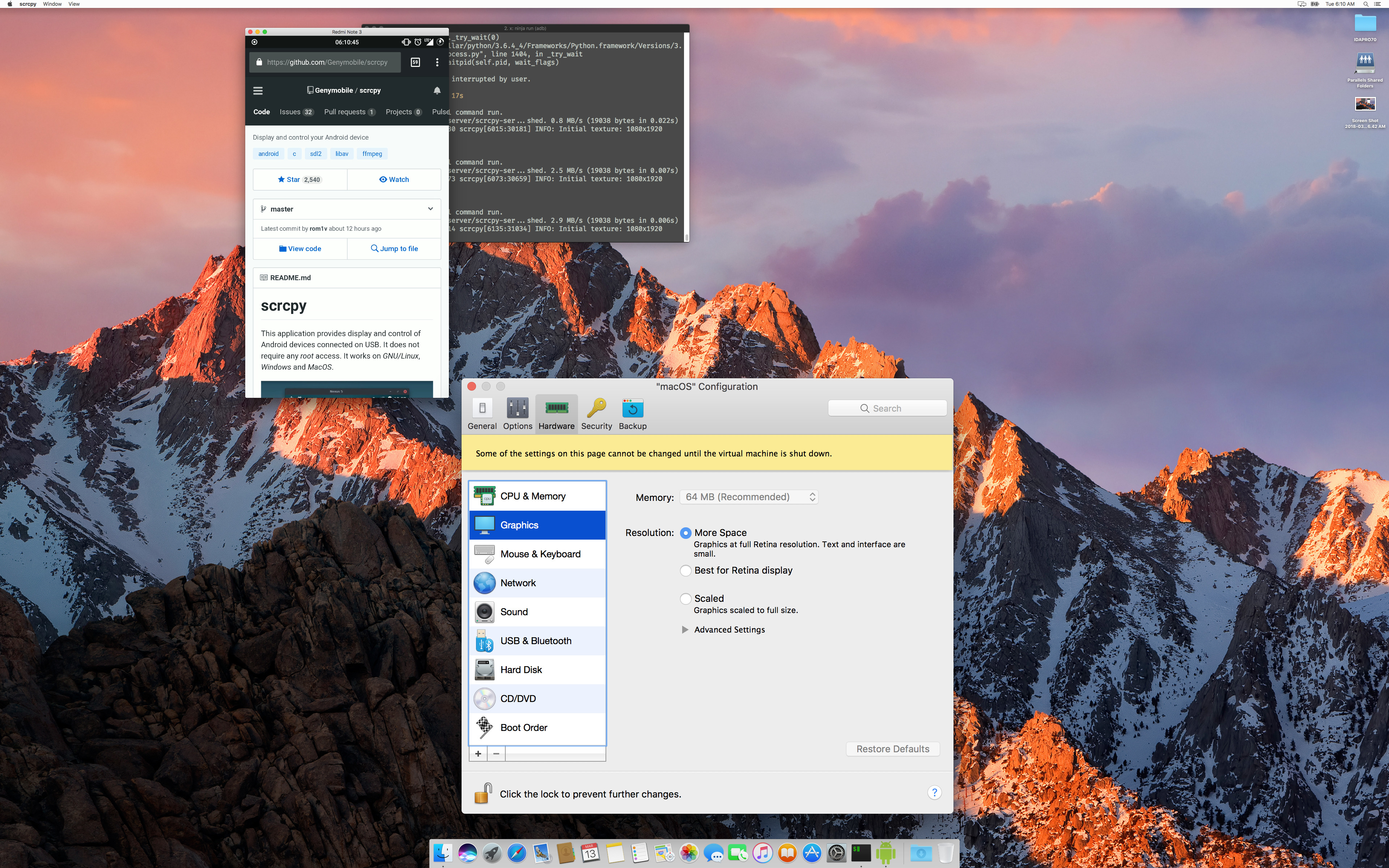
I don't know how to debug it. | bug,hidpi,macos | low | Critical |
304,661,311 | scrcpy | Add toggle button on window menu "stay on top" | As the title says.
| feature request,windows | low | Major |
304,695,135 | flutter | OnMemoryPressure is unreliable on Android | ## Steps to Reproduce
1. Create several cache's that load large amounts of data.
2. Create a handler for the OnMemoryPressure to clear the caches
3. Suspend application
4. Use another application (say: https://play.google.com/store/apps/details?id=me.empirical.android.application.fillmemory)
to fill memory
## Issue:
OnMemoryPressure is frequently triggered to late and the Flutter application is killed before it can clear the cache or even sometimes while it is clearing the cache.
## Relevant source:
This is the handlers that are captured from the Activity:
https://github.com/flutter/engine/blob/365627889410bbab8044528ad8e889881287a112/shell/platform/android/io/flutter/app/FlutterActivityDelegate.java#L266-L275
Both of them call:
https://github.com/flutter/engine/blob/5ce89a0f3deeb3b591a30de71351e3ec0120b4c5/shell/platform/android/io/flutter/view/FlutterView.java#L276-L280
Which sends a **Async** Message over to the Dart side AND then continues running.
The source of the call inside the Android OSP is:
https://github.com/aosp-mirror/platform_frameworks_base/blob/880839d2d10d0a3468a29b9ea20007159f014444/core/java/android/app/ActivityThread.java#L5311-L5331
If you notice right after it calls onLowMemory; on line 5331 it calls GC on the memory... This is so that is can then reclaim any memory freed.
Well, since it calls the kernel GC BEFORE we (the Flutter app) can actually cleanup; this leaves the Flutter application in a state where it now is considered for Termination because the GC happens more than likely before we can even sync the OnMemoryPressure event to our app. This depends on what is going on in the OS; if their are lots of apps running; the odds of our app surviving are improved; if there are not very many apps running and we are using a lot of memory; the odds of the OS terminating our Flutter app; because we are still using all that memory after a GC is greatly enhanced.
This would be fixed by Sync message (https://github.com/flutter/engine/pull/4358) support; since the return from the "OnTrimMemory" and "OnLowMemory" is what actually triggers the GC. If this even was Sync, we could clear our dart memory; return, and return to the OS which would then GC and consider our Flutter application a good citizen.
cc: @Hixie
| engine,c: performance,perf: memory,P3,team-engine,triaged-engine | high | Critical |
304,728,213 | pytorch | [feature request] Callback on learning rate drop in torch.optim.lr_scheduler.ReduceLROnPlateau | Currently [torch.optim.lr_scheduler.ReduceLROnPlateau](http://pytorch.org/docs/master/optim.html#torch.optim.lr_scheduler.ReduceLROnPlateau) has the option to print a fixed message once the learning rate drops.
I propose that either this be replaced, or modified in some way so that we can run an arbitrary closure / callback at each drop.
This could be useful for things such as logging which epochs the drop happened at, for terminating after a given number of drops, for taking a model snapshot, etc.
cc @vincentqb | todo,module: optimizer,triaged | low | Major |
304,762,154 | scrcpy | Add density controls | While it's nice that I can set `adb shell wm density XXX` before running scrcpy, it would be nicer to have this while using it, eg. CTRL-OP or something that increments density live (if it's possible).
If not live, maybe a start argument, that first sets density and then runs scrcpy. | feature request | low | Minor |
304,786,959 | pytorch | [feature request] scalar input to scatter_add_ like scatter_ | Note that #5659 only fix the bug reported in #5405 (thanks for @zou3519 pointing out, I open a new issue about that). Do we need scalar input to `scatter_add_`?
I think the implementation may be similar to `scatter_` where it broadcast the scalar based on `dim` and `index`. | triaged,function request,module: scatter & gather ops | low | Critical |
304,828,471 | vue | Globally registered component naming converts to kebap-case when PascalCase is used | ### Version
2.5.15
### Steps to reproduce
Currently all globally registered components automatically convert their name to kebap-case.
```js
// register component
Vue.component('RouterLink', {...})
// get component fails, returns undefined
Vue.component('RouterLink')
// get component works, returns the component
Vue.component('router-link')
```
It would be nice to have the registered components also available as with their PascalCase naming. This would also mean that you could use the PascalCase naming in `.vue` templates, which is a nice way to quickly distinguish Vue components from HTML elements. Right now its is not possible, and to make it work this code has to be used:
```html
<template>
<RouterLink :to="link">Link</RouterLink>
</template>
<script>
{
components: {
RouterLink: Vue.component('router-link')
}
}
</script>
```
### What is expected?
Access globally registered components with their PascalCase naming if it was provided on register function call.
### What is actually happening?
For now the component naming always converts to kebap-case.
<!-- generated by vue-issues. DO NOT REMOVE --> | improvement | medium | Minor |
304,866,202 | react | React onBlur events not firing during unmount | **Do you want to request a *feature* or report a *bug*?**
bug
**What is the current behavior?**
If a DOM element rendered by a React component has focus, and the React component unmounts, the React `onBlur` event does not fire on parent DOM elements.
**If the current behavior is a bug, please provide the steps to reproduce and if possible a minimal demo of the problem. Your bug will get fixed much faster if we can run your code and it doesn't have dependencies other than React. Paste the link to your JSFiddle (https://jsfiddle.net/Luktwrdm/) or CodeSandbox (https://codesandbox.io/s/new) example below:**
https://codesandbox.io/s/134wrzy6q7
**What is the expected behavior?**
I would expect that, just like the browser fires a `focusout` event when removing a DOM node, React would fire an `onBlur` events up to parent nodes when the focused node is removed / unmounted.
**Which versions of React, and which browser / OS are affected by this issue? Did this work in previous versions of React?**
React: 16.2
Mac OS X: 10.13.2
Browser: Chrome 67.0.3366.0, Chrome 64.0.3282.186
No idea if this worked in earlier versions of React. | Type: Bug,Component: DOM,Type: Needs Investigation | medium | Critical |
304,904,446 | godot | Input.is_action_just_released() not working with left/right shift and neo2 keyboard layout | **Godot version:**
3.0.2 official
**OS/device including version:**
Linux, Debian Stretch
**Issue description:**
Input.is_action_just_released() is not working for SHIFT if using neo 2 keyboard layout
tested successfully with standard "qwert" and "dvorak" layout, only "neo2" gives problems
| bug,platform:linuxbsd,topic:core,topic:input | low | Major |
304,939,149 | rust | Collecting into a Result<Vec<_>> doesn't reserve the capacity in advance | Couldn't find an issue for this and don't know if it counts but filing anyway.
----
If you have
fn foo(s: &[i8]) -> Vec<u8> {
s.iter()
.map(|&x| x as u8)
.collect()
}
the `SpecExtend` machinery ensures that the vector has `s.len()` space reserved in advance. However if you change it to return a result
fn foo(s: &[i8]) -> Result<Vec<u8>> {
s.iter()
.map(|&x| if x < 0 { Err(...) } else { Ok(x as u8) } )
.collect()
}
then (based on examining the LLVM IR and heaptracker's "Temporary" measurements) that optimization has quietly been lost.
This is technically correct in the sense that the first element yielded could be an `Err` of course (the size hint for the `Adapter` in `Result`'s `FromIterator` impl has a lower bound of 0). But this pessimizes the good path to take more memory and be slower in favor of possibly saving memory on the bad one, which seems backwards.
Is there a specialization that could be added to fix this? | I-slow,C-enhancement,T-libs,C-optimization | low | Major |
304,948,372 | go | x/sys/unix: add Solaris/Illumos ACL and extended attribute support | Would it be possible to get these calls added as part of Solaris/Illumos. Must use cgo to interface with file attributes otherwise. Some of these might exist, most don't. Most implementations seem to handle these via a syscall which we don't have in Solaris.
fgetattr,fsetattr,getattrat,setattrat
acl_get,facl_get,acl_set,facl_set, acl_totext, acl_fromtext,unlinkat
openat,fchownat,fstatat,futimesat,renameat, attropen, fdopendir, readdir, read, write
http://illumos.org/man/3lib/libc
http://illumos.org/man/3lib/libsec
### What version of Go are you using (`go version`)?
1.10
### Does this issue reproduce with the latest release?
Yes
### What operating system and processor architecture are you using (`go env`)?
amd64/solaris
### What did you do?
If possible, provide a recipe for reproducing the error.
A complete runnable program is good.
A link on play.golang.org is best.
### What did you expect to see?
Ability to change acls and extended attributes from syscall or os package.
### What did you see instead?
Need to use cgo.
| help wanted,OS-Solaris,NeedsFix,compiler/runtime | low | Critical |
304,955,615 | go | x/build/cmd/gerritbot: Gobot shouldn't be added as a CC/Reviewer | Gobot is added as a CC when it says "Trybots beginning", and as a Reviewer when it posts Trybot-Result+1 or Trybot-Result-1. For example, see: https://go-review.googlesource.com/c/go/+/100475
I realise that this is how Gerrit is supposed to work for humans - a user should be subscribed to updates if they comment, and added as a reviewer if they touch one of the flags/labels. However, I think this is noisy for bots. Is there a way to keep it from happening? | help wanted,Builders,NeedsFix | low | Major |
304,971,777 | flutter | libtxt: Single line RTL text is clipped on the beginning (right) of the text instead of the end (left). | When a single line of text (defined like this):
```dart
new Text('תווית ארוכה מאוד תווית ארוכה מאוד',
maxLines: 1,
softWrap: false,
overflow: TextOverflow.fade,
textAlign: TextAlign.start,
textDirection: TextDirection.rtl)
```
Reaches the end of its allowed size (e.g. it's placed inside a box that is constrained to a width smaller than the text box), it will clip as expected, but on RTL it will clip off the **beginning** of the string instead of the end.
I tried tracking this down, and got lost when it jumped to the platform side, but I know that it shows the same behavior on Blink and libtxt. I'm assuming it is somewhere in the ParagraphBuilder, or in Paragraph.layout. Looking at the ui.ParagraphStyle and the TextPainter, I couldn't see anything amiss.
For instance, the string above is clipped like this:

When the string should start (on the left) with "תווית", and the long string should be clipped on the right.
LTR strings clip properly, so it's clearly something to do with something not handling/knowing about the text direction. | framework,engine,a: internationalization,a: typography,has reproducible steps,P2,found in release: 3.3,found in release: 3.7,team-engine,triaged-engine | low | Major |
304,985,458 | godot | Editor plugin receives mouse events even if the Spatial gizmo is being manipulated | Godot 3.0.2
I made a plugin which lets the user paint on a terrain. However, I noticed that if I select the terrain node and try to drag the usual Spatial gizmo to move it, if my click lines up with the terrain, I end up painting and the gizmo doesn't reacts (because my plugin got the event and captured it).
If I don't capture the event, I end up both moving the gizmo, making a square selection and painting all at the same time, which get messy really quickly.
The gizmos should receive input first and capture it, before the event gets forwarded to the plugin. Or is there any other way to avoid this? | bug,topic:editor | low | Major |
304,987,006 | go | os/user: LookupUser() doesn't find users on macOS when compiled with CGO_ENABLED=0 | Please answer these questions before submitting your issue. Thanks!
### What version of Go are you using (`go version`)?
go version go1.9.2 darwin/amd64
### Does this issue reproduce with the latest release?
Yes.
### What operating system and processor architecture are you using (`go env`)?
GOARCH="amd64"
GOBIN=""
GOCACHE="/home/jeff_walter/.cache/go-build"
GOEXE=""
GOHOSTARCH="amd64"
GOHOSTOS="freebsd"
GOOS="darwin"
GOPATH="/home/jeff_walter/src/agent"
GORACE=""
GOROOT="/usr/local/go"
GOTMPDIR=""
GOTOOLDIR="/usr/local/go/pkg/tool/freebsd_amd64"
GCCGO="gccgo"
CC="clang"
CXX="clang++"
CGO_ENABLED="0"
CGO_CFLAGS="-g -O2"
CGO_CPPFLAGS=""
CGO_CXXFLAGS="-g -O2"
CGO_FFLAGS="-g -O2"
CGO_LDFLAGS="-g -O2"
PKG_CONFIG="pkg-config"
GOGCCFLAGS="-fPIC -m64 -fno-caret-diagnostics -Qunused-arguments -fmessage-length=0 -fdebug-prefix-map=/tmp/go-build053729832=/tmp/go-build -gno-record-gcc-switches -fno-common"
### What did you do?
If I run the following program with sudo I get a different result than I get when I run it as 'someuser' (where 'someuser' is my current logged in user).
```
package main
import (
"fmt"
"os/user"
)
func main() {
u, err := user.Lookup("someuser")
if err != nil {
fmt.Printf("%s", err)
return
}
fmt.Printf("%V", u)
}
```
### What did you expect to see?
$ ./user
&{%!V(string=502) %!V(string=20) %!V(string=someuser) %!V(string=) %!V(string=/Users/someuser)}
### What did you see instead?
$ sudo ./user
user: unknown user someuser
| OS-Darwin,NeedsInvestigation | medium | Critical |
305,000,301 | flutter | Merge debug tracing toggles | There are `debugProfilePaintsEnabled` and `debugProfileBuildsEnabled` to break down the timeline in debug mode for the framework build and paint phases. But for breaking down LayerTree::Paint in the GPU thread in the timeline, the toggle is on by passing `--trace-skia` when invoking the command line. Would be good to consolidate the debug toggles. | team,framework,engine,a: debugging,P3,team-engine,triaged-engine | low | Critical |
305,044,558 | nvm | upgrade node succeed but nodejs failed. | <!-- Thank you for being interested in nvm! Please help us by filling out the following form if you‘re having trouble. If you have a feature request, or some other question, please feel free to clear out the form. Thanks! -->
- Operating system and version:
Ubuntu server 14.04
- `nvm debug` output:
<details>
<!-- do not delete the following blank line -->
```sh
root@iZj6c0yo64w6enlu2ye50lZ:~# nvm debug
nvm --version: v0.33.8
$SHELL: /bin/bash
$HOME: /root
$NVM_DIR: '$HOME/.nvm'
$PREFIX: ''
$NPM_CONFIG_PREFIX: ''
$NVM_NODEJS_ORG_MIRROR: ''
$NVM_IOJS_ORG_MIRROR: ''
shell version: 'GNU bash, version 4.3.11(1)-release (x86_64-pc-linux-gnu)'
uname -a: 'Linux 4.4.0-79-generic #100~14.04.1-Ubuntu SMP Fri May 19 18:36:51 UTC 2017 x86_64 x86_64 x86_64 GNU/Linux'
OS version: Ubuntu 14.04.5 LTS
curl: /usr/bin/curl, curl 7.35.0 (x86_64-pc-linux-gnu) libcurl/7.35.0 OpenSSL/1.0.1f zlib/1.2.8 libidn/1.28 librtmp/2.3
wget: /usr/bin/wget, GNU Wget 1.15 built on linux-gnu.
git: /usr/bin/git, git version 2.14.2
grep: /bin/grep (grep --color=auto), grep (GNU grep) 2.16
awk: not an option: --version
awk: /usr/bin/awk,
sed: /bin/sed, sed (GNU sed) 4.2.2
cut: /usr/bin/cut, cut (GNU coreutils) 8.21
basename: /usr/bin/basename, basename (GNU coreutils) 8.21
rm: /bin/rm, rm (GNU coreutils) 8.21
mkdir: /bin/mkdir, mkdir (GNU coreutils) 8.21
xargs: /usr/bin/xargs, xargs (GNU findutils) 4.4.2
nvm current: v9.8.0
which node: $NVM_DIR/versions/node/v9.8.0/bin/node
which iojs:
which npm: $NVM_DIR/versions/node/v9.8.0/bin/npm
npm config get prefix: $NVM_DIR/versions/node/v9.8.0
npm root -g: $NVM_DIR/versions/node/v9.8.0/lib/node_modules
```
</details>
- `nvm ls` output:
<details>
<!-- do not delete the following blank line -->
```sh
root@iZj6c0yo64w6enlu2ye50lZ:~# nvm ls
-> v9.8.0
system
default -> node (-> v9.8.0)
node -> stable (-> v9.8.0) (default)
stable -> 9.8 (-> v9.8.0) (default)
iojs -> N/A (default)
lts/* -> lts/carbon (-> N/A)
lts/argon -> v4.8.7 (-> N/A)
lts/boron -> v6.13.1 (-> N/A)
lts/carbon -> v8.10.0 (-> N/A)
```
</details>
- How did you install `nvm`? (e.g. install script in readme, Homebrew):
script in readme
- What steps did you perform?
nvm install node
- What happened?
succeed.
```sh
root@iZj6c0yo64w6enlu2ye50lZ:~# node -v
v9.8.0
```
- What did you expect to happen?
`nodejs -v` should also return `v9.8.0`. but in fact it returns `v6.11.3`
- Is there anything in any of your profile files (`.bashrc`, `.bash_profile`, `.zshrc`, etc) that modifies the `PATH`?
No | OS: ubuntu | low | Critical |
305,081,183 | go | cmd/compile: keeping a subset of a struct value's fields is slow | ### What version of Go are you using (`go version`)?
go version devel +e601c07908 Tue Mar 13 22:02:46 2018 +0000 linux/amd64
### What did you do?
https://play.golang.org/p/H9LaiI3BP-U
See the comments as to why I prefer writing the slower version, as it helps maintain the software in the long run.
### What did you expect to see?
The two to perform the same, or at least comparably.
### What did you see instead?
The second method being easily 10x as slow.
BenchmarkResetUnwanted-4 2000000000 0.72 ns/op 0 B/op 0 allocs/op
BenchmarkKeepWanted-4 200000000 9.72 ns/op 0 B/op 0 allocs/op
I can imagine why this is; naively, the second function does more work. It has to write the entire struct - 7 ints - instead of just three ints, like the first one. However, I'd hope that the compiler can learn to recognise assignments like these to avoid the unnecessary extra work. | Performance,compiler/runtime | low | Major |
305,124,139 | neovim | swapfile: support long file names | <!-- Before reporting: search existing issues and check the FAQ. -->
- `nvim --version`: v0.2.2
- Vim (version: 8.0) behaves differently? Yes
- Operating system/version: Arch Linux
- Terminal name/version: GNOME Terminal 3.26.2 Using VTE version 0.50.3 +GNUTLS
- `$TERM`: xterm-256color
- [strace.log](https://github.com/neovim/neovim/files/1808572/strace.log)
### Details
NVim directory:
:set directory?
directory=~/.local/share/nvim/swap//
Debugging:
$ ls -al ~/.local/share/nvim/swap//
total 120
drwxr-xr-x 2 kevin kevin 4096 Mar 14 08:16 .
drwx------ 5 kevin kevin 4096 Nov 9 02:08 ..
$ strace -o strace.log nvim -u NONE my_file
$ grep ENAMETOOLONG strage.log
lstat("/home/kevin/.local/share/nvim/swap//%home%kevin%olivia%olivia_git%data_manager%olivia_modules%cashflow-pattern-detector%rules-historical-cashflow%similar-transaction-count-heuristics.js.swp", 0x7ffc674c52d0) = -1 ENAMETOOLONG (File name too long)
lstat("/home/kevin/.local/share/nvim/swap//%home%kevin%olivia%olivia_git%data_manager%olivia_modules%cashflow-pattern-detector%rules-historical-cashflow%similar-transaction-count-heuristics.js.swp", 0x7ffc674c57e0) = -1 ENAMETOOLONG (File name too long)
openat(AT_FDCWD, "/home/kevin/.local/share/nvim/swap//%home%kevin%olivia%olivia_git%data_manager%olivia_modules%cashflow-pattern-detector%rules-historical-cashflow%similar-transaction-count-heuristics.js.swp", O_RDWR|O_CREAT|O_EXCL|O_NOFOLLOW|O_CLOEXEC, 0600) = -1 ENAMETOOLONG (File name too long)
I've found very strange the strace log, because from what I've seen, the `ENAMETOOLONG` error should be bounded to the `NAME_MAX` variable, which is equal to 255 in my system, and the path does not exceed this value.
Can anyone confirm if this is the source of the NeoVim error? Here is the complete strace log:
https://github.com/neovim/neovim/files/1808572/strace.log | enhancement,bug-vim | low | Critical |
305,152,203 | vue | v-model support for web components (stenciljs) | ### What problem does this feature solve?
V-model support for web components(tested with web component implemented with ionic's stenciljs compiler).
Does not work:
```
<ui-input v-model="mySelect" />
```
Works:
```
<ui-input :value="mySelect" @input="mySelect = $event.target.value" />
```
Can this be enabled to support ignored elements as well that have been declared with:
```Vue.config.ignoredElements = [/^ui-/];```
### What does the proposed API look like?
Declaration
```
Vue.config.ignoredElements = [/^ui-/];
```
Usage
```
<ui-input v-model="mySelect" />
```
<!-- generated by vue-issues. DO NOT REMOVE --> | feature request | medium | Critical |
305,195,743 | rust | Incorrect recursion in type inference | I encountered a recursion overflow error on a trait implementation of HashMap (`Encodable`) when I tried to extend an unrelated trait (`Inner`) by a where clause for the same trait. This might end in a case of 'You are holding it wrong', but I couldn't find any clue why the change should infer with the given implementation:
Minimal Example
---------
[Playground](https://play.rust-lang.org/?gist=c0f644997edc02c89ee81eff442c9ed3&version=stable)
```rust
use std::collections::HashMap;
pub trait Encodable {}
pub trait Inner
where
for<'a> &'a Self: Encodable,
{
}
pub trait Outer {
type Inner: Inner;
}
impl<'a, K, V, S> Encodable for &'a HashMap<K, V, S>
where
&'a V: Encodable,
{
}
```
Error Log
------
```error
Compiling playground v0.0.1 (file:///playground)
error[E0275]: overflow evaluating the requirement `_: std::marker::Sized`
|
= help: consider adding a `#![recursion_limit="128"]` attribute to your crate
= note: required because of the requirements on the impl of `for<'a> Encodable` for `&'a std::collections::HashMap<_, _, _>`
= note: required because of the requirements on the impl of `for<'a> Encodable` for `&'a std::collections::HashMap<_, std::collections::HashMap<_, _, _>, _>`
= note: required because of the requirements on the impl of `for<'a> Encodable` for `&'a std::collections::HashMap<_, std::collections::HashMap<_, std::collections::HashMap<_, _, _>, _>, _>`
= note: required because of the requirements on the impl of `for<'a> Encodable` for `&'a std::collections::HashMap<_, std::collections::HashMap<_, std::collections::HashMap<_, std::collections::HashMap<_, _, _>, _>, _>, _>`
= note: required because of the requirements on the impl of `for<'a> Encodable` for `&'a std::collections::HashMap<_, std::collections::HashMap<_, std::collections::HashMap<_, std::collections::HashMap<_, std::collections::HashMap<_, _, _>, _>, _>, _>, _>`
[...]
```
Reproduced with:
- stable: rustc 1.24.1 (d3ae9a9e0 2018-02-27)
- nightly: rustc 1.26.0-nightly (2789b067d 2018-03-06) | A-trait-system,T-compiler,C-bug | low | Critical |
305,247,831 | flutter | Slow scrolling on ios when the scroll view or any of it's children contains an image. | Scrolling is super slow and choppy when it or any of it's children contain and image. No matter where I initialize the image (as a method variable, directly in the Widget, or even if I initialize it outside of the method and just pass it in as a parameter) which leads me to believe that it has to do with fetching the image (in this case it's provided not fetched over a network). This doesn't happen on the android emulator, but it does happen on the iOS simulator (all models) and an actual iPhone 6. However i wasn't as bad when i tested on the iOS simulator but it was still awful.
```dart
Widget sidePager(BuildContext context, String imageUrl) {
final _width = MediaQuery.of(context).size.width;
final _height = 125.0;
final _image = new Image.asset(imageUrl);
return new SingleChildScrollView(
scrollDirection: Axis.horizontal,
child: new Row(
children: <Widget>[
new Container(
height: _height,
width: _width,
color: Colors.blue.withOpacity(0.3),
child: new Container(
height: _height,
width: _height,
decoration: new BoxDecoration(
shape: BoxShape.circle,
border: new Border.all(color: Colors.white, width: 1.0),
),
child: new ClipOval(
// TODO: Figure out why scrolling is choppy and slow when image is used
child: _image,
),
),
),
new Container(
height: _height,
width: _width,
color: Colors.orange.withOpacity(0.3),
child: new CupertinoButton(child: new Text("Enter Lockdown"), onPressed: presentLockdownView)),
],
),
);
}
``` | e: device-specific,platform-ios,engine,c: performance,dependency: skia,a: images,has reproducible steps,P2,team-engine,triaged-engine,found in release: 3.13,found in release: 3.15 | low | Major |
305,263,765 | TypeScript | Recursive conditional types are aliased |
I am trying to create a conditional type that converts
```ts
type Before = {
a: string;
b: number;
c: string | undefined;
d: number | undefined;
nested: {
a2: string;
b2: number;
c2: string | undefined;
d2: number | undefined;
nested2: {
a3: string;
b3: number;
c3: string | undefined;
d3: number | undefined;
};
};
};
```
to
```ts
type After = {
a: string;
b: number;
c?: string | undefined;
d?: number | undefined;
nested: {
a2: string;
b2: number;
c2?: string | undefined;
d2?: number | undefined;
nested2: {
a3: string;
b3: number;
c3?: string | undefined;
d3?: number | undefined;
};
};
};
```
When I hover on `After` in the below code
```ts
const fnBefore = (input: Before) => {
return input;
};
const fnAfter = (input: After) => {
return input;
};
```
it shows
```ts
type After = {
c?: string | undefined;
d?: number | undefined;
a: string;
b: number;
nested: Flatten<{
c2?: string | undefined;
d2?: number | undefined;
} & {
a2: string;
b2: number;
nested2: Flatten<{
c3?: string | undefined;
d3?: number | undefined;
} & RequiredProps>;
}>;
}
```
instead of properly converted type.
According to #22011
> If an conditional type is instantiated over 100 times, we consider that to be too deep.
At that point, we try to find the respective alias type that contains that conditional type.
only more complex types should be aliased, but I always face this issue.
Also for simple types:
```ts
type Simple = {
nested: {
a2: string;
c2: string | undefined;
};
};
```
**TypeScript Version:** 2.8.0-dev.201180314
**Full Code**
```ts
type Before = {
a: string;
b: number;
c: string | undefined;
d: number | undefined;
nested: {
a2: string;
b2: number;
c2: string | undefined;
d2: number | undefined;
nested2: {
a3: string;
b3: number;
c3: string | undefined;
d3: number | undefined;
};
};
};
type Simple = {
nested: {
a2: string;
c2: string | undefined;
};
};
type Flatten<T> = { [K in keyof T]: T[K] };
type OptionalPropNames<T> = { [P in keyof T]: undefined extends T[P] ? P : never }[keyof T];
type RequiredPropNames<T> = { [P in keyof T]: undefined extends T[P] ? never : P }[keyof T];
type OptionalProps<T> = { [P in OptionalPropNames<T>]: T[P] };
type RequiredProps<T> = { [P in RequiredPropNames<T>]: T[P] };
type MakeOptional<T> = { [P in keyof T]?: T[P] };
type ConvertObject<T> = Flatten<MakeOptional<OptionalProps<T>> & RequiredProps<T>>;
type DeepConvertObject<T> = ConvertObject<{ [P in keyof T]: DeepConvert<T[P]> }>;
type DeepConvert<T> = T extends object ? DeepConvertObject<T> : T;
type After = DeepConvert<Before>;
type SimpleAfter = DeepConvert<Simple>;
const fnBefore = (input: Before) => {
return input;
};
const fnAfter = (input: After) => {
return input;
};
```
**Expected behavior:**
The tooltip should be shown without aliases.
**Actual behavior:**
The tooltip is shown with aliases.
| Suggestion,Experience Enhancement | low | Major |
305,301,701 | go | runtime: panic: runtime error: index out of range on all commands on Darwin | Please answer these questions before submitting your issue. Thanks!
### What version of Go are you using (`go version`)?
$ go version
panic: runtime error: index out of range
goroutine 1 [running]:
os.executable(...)
/usr/local/Cellar/go/1.10/libexec/src/os/executable_darwin.go:13
os.Executable(...)
/usr/local/Cellar/go/1.10/libexec/src/os/executable.go:21
cmd/go/internal/cfg.findGOROOT(0xc42001e2c0, 0x17)
/usr/local/Cellar/go/1.10/libexec/src/cmd/go/internal/cfg/cfg.go:107 +0x441
### Does this issue reproduce with the latest release?
I don't know what version, I think 1.10 based on the .pkg download
### What operating system and processor architecture are you using (`go env`)?
OSX 10.12
$ go env
panic: runtime error: index out of range
goroutine 1 [running]:
os.executable(...)
/usr/local/Cellar/go/1.10/libexec/src/os/executable_darwin.go:13
os.Executable(...)
/usr/local/Cellar/go/1.10/libexec/src/os/executable.go:21
cmd/go/internal/cfg.findGOROOT(0xc42001e2c0, 0x17)
/usr/local/Cellar/go/1.10/libexec/src/cmd/go/internal/cfg/cfg.go:107 +0x441
### What did you do?
Installed go.
Let me know if you need any other docs.
Any idea why it isn't working?
| OS-Darwin,NeedsInvestigation | low | Critical |
305,305,991 | flutter | Clearer error message for crash when mixing 64 and 32 bit code on Android | According to @jason-simmons, on 64-bit Android devices, Flutter will automatically build with 64-bit native code but it's possible to bring in other 32-bit JNI code via gradle. Launching an app built this way would crash on launch.
```
03-14 12:49:37.531 4779 4779 D AndroidRuntime: Shutting down VM
03-14 12:49:37.531 4779 4779 E AndroidRuntime: FATAL EXCEPTION: main
03-14 12:49:37.531 4779 4779 E AndroidRuntime: Process: com.hamilton.app, PID: 4779
03-14 12:49:37.531 4779 4779 E AndroidRuntime: java.lang.UnsatisfiedLinkError: dalvik.system.PathClassLoader[DexPathList[[zip file "/data/app/com.hamilton.app-2/base.apk"],nativeLibraryDirectories=[/data/app/com.hamilton.app-2/lib/arm, /data/app/com.hamilton.app-2/base.apk!/lib/armeabi-v7a, /system/lib, /vendor/lib]]] couldn't find "libflutter.so"
03-14 12:49:37.531 4779 4779 E AndroidRuntime: at java.lang.Runtime.loadLibrary0(Runtime.java:972)
03-14 12:49:37.531 4779 4779 E AndroidRuntime: at java.lang.System.loadLibrary(System.java:1567)
03-14 12:49:37.531 4779 4779 E AndroidRuntime: at io.flutter.view.FlutterMain.startInitialization(FlutterMain.java:163)
03-14 12:49:37.531 4779 4779 E AndroidRuntime: at io.flutter.view.FlutterMain.startInitialization(FlutterMain.java:148)
03-14 12:49:37.531 4779 4779 E AndroidRuntime: at io.flutter.app.FlutterApplication.onCreate(FlutterApplication.java:20)
03-14 12:49:37.531 4779 4779 E AndroidRuntime: at com.hamilton.app.App.onCreate(App.kt:16)
03-14 12:49:37.531 4779 4779 E AndroidRuntime: at android.app.Instrumentation.callApplicationOnCreate(Instrumentation.java:1032)
03-14 12:49:37.531 4779 4779 E AndroidRuntime: at android.app.ActivityThread.handleBindApplication(ActivityThread.java:5970)
03-14 12:49:37.531 4779 4779 E AndroidRuntime: at android.app.ActivityThread.-wrap3(ActivityThread.java)
03-14 12:49:37.531 4779 4779 E AndroidRuntime: at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1710)
03-14 12:49:37.531 4779 4779 E AndroidRuntime: at android.os.Handler.dispatchMessage(Handler.java:102)
03-14 12:49:37.531 4779 4779 E AndroidRuntime: at android.os.Looper.loop(Looper.java:154)
03-14 12:49:37.531 4779 4779 E AndroidRuntime: at android.app.ActivityThread.main(ActivityThread.java:6776)
03-14 12:49:37.531 4779 4779 E AndroidRuntime: at java.lang.reflect.Method.invoke(Native Method)
03-14 12:49:37.531 4779 4779 E AndroidRuntime: at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:1520)
03-14 12:49:37.531 4779 4779 E AndroidRuntime: at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:1410)
```
If possible, detect and adapt automatically while building.
The current solution according to Jason is to build on the command-line and switch everything to 32-bit by building with something like `flutter run --target-platform android-arm`.
cc @devoncarew since this workaround doesn't seem to be available in IntelliJ. | c: crash,platform-android,tool,customer: posse (eap),t: gradle,P2,team-android,triaged-android | low | Critical |
305,323,585 | pytorch | Add hookable weights | It would be nice to have hookable weights, where per-layer parameters can be operated upon and determined dynamically during each forward pass. The following is a short list of techniques that could benefit from this functionality:
- Quantization[[1]](https://arxiv.org/abs/1609.07061)
- Pruning[[2]](https://arxiv.org/abs/1510.00149)[[3]](https://arxiv.org/abs/1707.06168)[[4]](https://arxiv.org/abs/1802.00124)
- Stochastic gradient estimation[[5]](https://arxiv.org/abs/1711.00123)[[6]](https://arxiv.org/abs/1703.07370)
- DropConnect[[7]](https://cs.nyu.edu/~wanli/dropc/)
- Regularization[[8]](https://openreview.net/forum?id=H1Y8hhg0b)
- Manifold Tangent Classifier[[9]](https://papers.nips.cc/paper/4409-the-manifold-tangent-classifier.pdf)
I've begun work on [such a framework](https://github.com/castorini/candle), but the code is research-oriented and not production ready. I think the community would benefit from having official support for hookable weights.
I think it would also be interesting to have a more extensible backprop framework, in the sense of allowing for computation of different user-defined quantities across the computation graph. For example, the Hessian diagonals can be computed efficiently[[10]](http://yann.lecun.com/exdb/publis/pdf/lecun-90b.pdf) using a backprop-like approach.
Thanks for reading.
cc @albanD @mruberry @jbschlosser @jerryzh168 @jianyuh @dzhulgakov @raghuramank100 @jamesr66a @vkuzo | module: nn,triaged,enhancement | medium | Major |
305,377,029 | rust | improve wording of "possible candidate is found in another module" with enums | When compiling:
```rust
enum E { Foo }
fn main() {
println!("{}", Foo);
}
```
rustc currently produces:
```rust
error[E0425]: cannot find value `Foo` in this scope
--> src/main.rs:3:20
|
3 | println!("{}", Foo);
| ^^^ not found in this scope
help: possible candidate is found in another module, you can import it into scope
|
1 | use E::Foo;
|
```
This is a great error message except for one thing: the enum `E` is not really a "module" (even if it behaves like one for scoping purposes), so saying the candidate is "in another module" can be confusing. It would be better to modify the wording in this case. | A-frontend,C-enhancement,A-diagnostics,T-compiler,WG-diagnostics | low | Critical |
305,378,793 | go | plugin: type switches fail with reflect-created types | cmd/compile generates type hashes using MD5, but package reflect uses FNV-1 when dynamically constructing anonymous types. This causes type switches (which first search on type hash) to misbehave when using package plugin:
$ go build -buildmode=plugin w.go
$ go run x.go
FAIL; got *[8675309]int
$ cat x.go
package main
import (
"plugin"
"reflect"
)
func main() {
v := reflect.New(reflect.ArrayOf(8675309, reflect.TypeOf(0))).Interface()
p, err := plugin.Open("w.so")
if err != nil {
panic(err)
}
f, err := p.Lookup("F")
if err != nil {
panic(err)
}
f.(func(interface{}))(v)
}
$ cat w.go
package main
import "fmt"
func F(x interface{}) {
switch x.(type) {
case *[8675309]int:
fmt.Println("ok")
default:
fmt.Printf("FAIL; got %T\n", x)
}
}
Notably, the failure goes away if the array type is constructed after the plugin.Open call, or if there's an explicit `*[8675309]int` type in x.go.
/cc @ianlancetaylor @mwhudson @crawshaw | help wanted,NeedsFix,compiler/runtime | low | Critical |
305,384,398 | rust | panic mode where it aborts upon panic iff there are no catch_unwind calls to catch it | The two options for panic handling, abort and unwind, seem insufficient to cover my use case. When I release my app to users, I use a crash reporter (catches crash signals with a signal handler, records the stack trace, and uploads it). If I use abort for panics, then I'll see the stack trace of the panic in my crash reporter, but I won't be able to catch panics. If I use unwind, then I'll be able to catch panics, but uncaught panics will unwind all the way to the FFI boundary (this is an app built in another language and calls into Rust). This means that I won't be able to see the full stack trace. I see a few existing solutions:
- Use abort mode and never catch panics (this won't work for me personally, because there are places where I need to catch panics, e.g. for OOM)
- Have a thread-local variable with the number of catch_unwind calls that I'm currently nested in. Use my own version of catch_unwind to update it. If this number is 0, then that means the panic is going to abort after some amount of unwinding, so just abort it right there. Otherwise, leave it alone because it will be caught gracefully. This will cause aborts if the catch_unwind calls are outside of my code base, but that doesn't seem too common
Any ideas what a good way to deal with this is? | A-codegen,T-lang,C-feature-request | medium | Critical |
305,406,539 | rust | Possible Vec::split improvement | Hi guys! The other day I was working on something where I had some ``Vec<u8>`` data and wanted to split on two consecutive bytes. Normally with strings I can just do:
```rust
let v: Vec<&str> = "Hello\r\nWorld!".split("\r\n").collect();
```
But for ``Vec<u8>`` it only accepts a closure rather than a Pattern type, so it's not very easy to split on consecutive bytes. I noticed that ``String::split`` coerces closures and strings into Patterns that it uses to do the split. Do you think it would be possible (or even a good idea) to modify ``Vec::split`` and ``slice::split`` to accept any Pattern type and implement a new searcher for any PartialEq type? That would be pretty nice. Then we could do things like:
```rust
let v: Vec<u8> = b"Hello\r\nWorldWithOtherNonUtf8Stuff".split(b"\r\n").collect();
```
Which is useful if you're dealing with lots of bytes that may or may not be valid UTF-8.
And just generally it makes sense to me that if ``String::split`` accepts a ``&str``, that ``Vec<T>::split`` would accept a ``&[T]``.
Maybe I'm missing something obvious that makes this impossible since I imagine someone would've done it already if it were possible. | T-libs-api,C-feature-request | low | Major |
305,413,238 | flutter | Document widget creation source in Timeline RenderObject and Layers | With debugProfilePaintsEnabled and --trace-skia turned on, a nice RenderObject.paint call stack and Layer stack on the engine is printed. But it's hard to figure out where they are and where they came from.
If possible reuse widget inspector magic to tag these Timeline entries with args to show its source code widget creation place. | c: new feature,framework,d: api docs,f: inspector,a: debugging,P3,team-framework,triaged-framework | low | Critical |
305,414,003 | flutter | Create a debug rebuild rainbow debug overlay like the repaint rainbow? | c: new feature,framework,a: debugging,P3,team-framework,triaged-framework | low | Critical |
|
305,426,465 | flutter | If downloading dart sdk fails, cache is left in a bad state: FileSystemException: Cannot open file, path = '.../flutter/bin/cache/dart-sdk/version' | Get a new flutter version, run flutter, ctrl-c as soon as Downloading Dart SDK from Flutter engine xxxx... appears.
I ended up in a state where
```
Building flutter tool...
Unhandled exception:
FileSystemException: Cannot open file, path = '.../flutter/bin/cache/dart-sdk/version' (OS Error: No such file or directory, errno = 2)
#0 _File.throwIfError (dart:io/file_impl.dart:628)
#1 _File.openSync (dart:io/file_impl.dart:472)
#2 _File.readAsBytesSync (dart:io/file_impl.dart:532)
#3 _File.readAsStringSync (dart:io/file_impl.dart:577)
#4 readTextFile (package:pub/src/io.dart:153)
#5 _getVersion (package:pub/src/sdk.dart:44)
#6 version (package:pub/src/sdk.dart:32)
#7 version (package:pub/src/sdk.dart:32)
#8 PubCommandRunner.runCommand (package:pub/src/command_runner.dart:159)
<asynchronous suspension>
#9 PubCommandRunner.run (package:pub/src/command_runner.dart:116)
<asynchronous suspension>
#10 main (file:///b/build/slave/Mac_Engine/build/src/third_party/dart/third_party/pkg/pub/bin/pub.dart:8)
#11 _startIsolate.<anonymous closure> (dart:isolate-patch/dart:isolate/isolate_patch.dart:277)
#12 _RawReceivePortImpl._handleMessage (dart:isolate-patch/dart:isolate/isolate_patch.dart:165)
Error: Unable to pub upgrade flutter tool. Retrying in five seconds...
Unhandled exception:
FileSystemException: Cannot open file, path = '.../flutter/bin/cache/dart-sdk/version' (OS Error: No such file or directory, errno = 2)
#0 _File.throwIfError (dart:io/file_impl.dart:628)
#1 _File.openSync (dart:io/file_impl.dart:472)
#2 _File.readAsBytesSync (dart:io/file_impl.dart:532)
#3 _File.readAsStringSync (dart:io/file_impl.dart:577)
#4 readTextFile (package:pub/src/io.dart:153)
#5 _getVersion (package:pub/src/sdk.dart:44)
#6 version (package:pub/src/sdk.dart:32)
#7 version (package:pub/src/sdk.dart:32)
#8 PubCommandRunner.runCommand (package:pub/src/command_runner.dart:159)
<asynchronous suspension>
#9 PubCommandRunner.run (package:pub/src/command_runner.dart:116)
<asynchronous suspension>
#10 main (file:///b/build/slave/Mac_Engine/build/src/third_party/dart/third_party/pkg/pub/bin/pub.dart:8)
#11 _startIsolate.<anonymous closure> (dart:isolate-patch/dart:isolate/isolate_patch.dart:277)
#12 _RawReceivePortImpl._handleMessage (dart:isolate-patch/dart:isolate/isolate_patch.dart:165)
Error: Unable to pub upgrade flutter tool. Retrying in five seconds...
```
permanently until I `rm -rf bin/cache` | c: crash,tool,P2,team-tool,triaged-tool | low | Critical |
305,428,661 | flutter | "Google Calendar" AppBar animation | ## What I'm trying to do:
I am trying to build an AppBar in my app that can expand with an animation programmatically - not using a SliverAppBar with CustomScrollView and nested scrolling. The most similar example that I can think of is when you tap the month in Google Calendar, the app bar expands to show a child "month" view.
## Basic Scenario:
My goal is that the AppBar has height "h1" as its default state, and when an action is tapped - it uses an animation to expand to height "h2".
## My High-Level Design:
I have tried a few things, but strictly subclassing AppBar doesn't seem like a good option because the PreferredSizeWidget is rigid in height. A more ideal scenario seems to be using SliverAppBar within a Scaffold that has flexible space and having a defined initial height and a calculated height to "grow" to. I'm not worried about the animation part, but more-so just the basic skeleton design. Using an AppBar is preferred to show title, actions, and other provided flutter AppBar behavior.
If anyone has any ideas or can point me to similar examples, it would help me out quite a bit! | c: new feature,framework,a: animation,f: material design,c: proposal,P2,team-design,triaged-design | low | Minor |
305,545,292 | godot | [Bullet] 3D StaticBody constant linear/angular velocity doesn't work when using Bullet | **Godot version:** 3.0.2
**Issue description:**
While building my first game to test Godot out, I was [reading about StaticBody](https://godot.readthedocs.io/en/latest/classes/class_staticbody.html#class-staticbody)s in the documentation and stumbled upon constant velocity:
> [...] a constant linear or angular velocity can be set for the static body, so even if it doesn’t move, it affects other bodies as if it was moving [...]
It turns out, these properties do not have any effect at all with Bullet (the default 3D physics engine in Godot 3). With the engine set to `GodotPhysics`, these properties work as expected.
I need to investigate a bit further, but it seems the same thing is true for the thing the documentation calls "simulated motion mode".
**Steps to reproduce:**
Create a new scene with a StaticBody (e.g. a floor) and a RigidBody (e.g. a ball) and let the RigidBody drop on the StaticBody. Set `constant_linear_velocity` or `constant_angular_velocity` to any amount.
The RigidBody will be launched when `3d/physics_engine="GodotPhysics"`, but won't do anything for any other setting.
**Minimal reproduction project:**
Standalone test scenes are in this repository:
https://github.com/eiszfuchs/godot-test-scenes/blob/master/Scenes/ConstantLinearVelocity.tscn
https://github.com/eiszfuchs/godot-test-scenes/blob/master/Scenes/ConstantAngularVelocity.tscn | bug,confirmed,topic:physics | low | Major |
305,550,809 | angular | Feature Request: Automatic Mock Components | # The Problem
In unit testing components, one has to declare all child components (and their children, recursively) and directives in the call to `TestBed.configureTestingModule()`. For simple components which do not have many child components, this is okay.
The problem occurs when testing a component which has many descendant components. Consider the following component dependency graph:
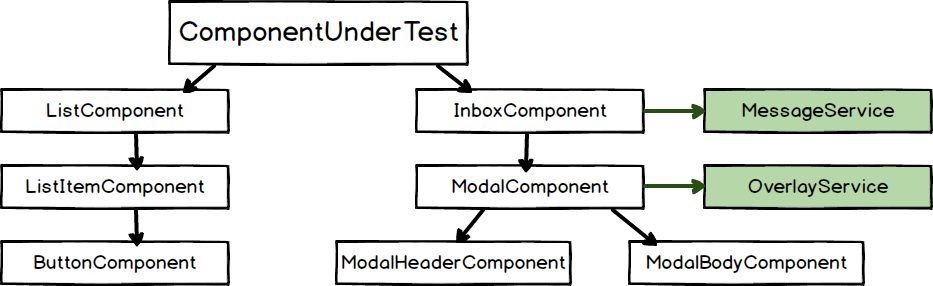
If I want to write a unit test for `ComponentUnderTest`, I'll have to declare my testing module like this:
```TypeScript
TestBed.configureTestingModule({
declarations: [
ComponentUnderTest,
ListComponent,
ListItemComponent,
ButtonComponent,
InboxComponent,
ModalComponent,
ModalHeaderComponent,
ModalFooterComponent
],
providers: [
MessageService,
OverlayService
]
})
```
This is already getting out of hand, and in reality things can get much more involved (the transitive dependencies of the services, for example).
# Current Solutions
Generally when I run into this I do one of 2 things, both of which are unsatisfactory:
1. Say "sod it!" and use `NO_ERRORS_SCHEMA`.
2. Manually mock the first-order dependencies (`ListComponent` and `InboxComponent` in the above example)
The first solution is not ideal because it renders the tests brittle - e.g. if you rename an input of `InboxComponent` but forget to update the template in the component under test, this will not be picked up.
The second solution can become a pain since you also need to set up all Inputs, Outputs and methods which are being used by the component under test. This leads to inflated spec files, repetition and more brittle tests once again.
# Proposal: Automatic Mocking
A solution which is both developer-friendly and robust would be to expose a feature (a function or decorator from `@angular/core/testing`) which takes a component class and returns a mock class with the correct selector, Inputs, Outputs and stubbed methods.
Here is how it might be used:
```TypeScript
import {TestBed, mockComponent} from '@angular/core/testing';
class MockListComponent extends mockComponent(ListComponent) {}
class MockInboxComponent extends mockComponent(InboxComponent) {}
describe('ComponentUnderTest', () => {
beforeEach(() => {
TestBed.configureTestingModule({
declarations: [
ComponentUnderTest,
MockListComponent,
MockInboxComponent
]
});
});
it('does stuff with InboxComponent', () => {
const fixture = TestBed.createComponent(ComponentUnderTest);
const inboxComponent: MockInboxComponent = fixture.debugElement.query(By.directive(MockInboxComponent)).componentInstance;
// We can now make assertions on the methods of InboxComponent
expect(inboxComponent.someMethod).not.toHaveBeenCalled();
// We can also emit events from InboxComponent and test that ComponentUnderTest
// reacts to them in the expected way.
inboxComponent.someEvent.emit('foo');
});
]);
```
## Advantages
1. No longer need to declare the entire dependency graph, only the first-order dependencies need to be mocked.
2. The mocks created by `mockComponent()` would be type-safe, so you get auto-completion on public properties (inputs, outputs, public methods).
3. The mocks are generated from the real component, so e.g. changes to an input of `InboxComponent` would raise errors when trying to run the test if the template of `ComponentUnderTest`. This mitigates potential brittleness introduced by hand-coded mock component.
4. Much less boilerplate code than either declaring all dependencies or manually mocking.
## Implementation
I wrote a proof-of-concept implementation which can be found here: https://gist.github.com/michaelbromley/bb4291200c25196507d12d2fd948a13e
I also discovered an independently-developed solution which does essentially the same thing: https://github.com/ike18t/mock_component
## Limitations
There are 2 main issues I am aware of with the above implementations:
1. They rely on internal data structures for reading the annotations and property metadata from the target component (e.g. my implementation reads the selector like this: `const selector = (original as any).__annotations__[0].selector;` . This makes the solution unstable and liable to break with changes to Angular internals.
2. I could not yet get this to work when the `ComponentUnderTest` uses a query of the type `@ViewChild(InboxComponent)`. I'm sure there must be a way to get this to work with more digging.
## Proposal
Due to limitation 1 above, there seems no way to implement such functionality *safely* in user-land. So I would like to see Angular ship with a built-in helper which implements something like `mockComponent()` in a safe, forward-compatible way.
---
Thanks for your time! | feature,area: testing,freq2: medium,feature: under consideration | medium | Critical |
305,555,443 | opencv | camera led stays on after release | When I release my logitech c920, the led goes off but in one second it goes on again. it happens 1 from 4 times probably, when i try the most simple example, the led stays on (probability is about 20%)
##### System information (version)
- OpenCV => 3.4
- Operating System / Platform => Windows 7 64 Bit
- Compiler => Visual Studio 2017
[version_string.txt](https://github.com/opencv/opencv/files/1815514/version_string.txt)
I tried vfw and dshow backends, the same behaviour for both
The led stays on even when the app exited.
Even the most simple example does this
.pro file
-------------------
QT -= gui
CONFIG += c++11 console
CONFIG -= app_bundle
DEFINES += QT_DEPRECATED_WARNINGS
#DEFINES += QT_DISABLE_DEPRECATED_BEFORE=0x060000 # disables all the APIs deprecated before Qt 6.0.0
SOURCES += main.cpp
CONFIG(debug, release|debug) {
OPENCV_CORE = opencv_core340d
OPENCV_HIGHGUI = opencv_highgui340d
OPENCV_IMGPROC = opencv_imgproc340d
OPENCV_VIDEO = opencv_video340d
OPENCV_VIDEOIO = opencv_videoio340d
} else {
OPENCV_CORE = opencv_core340
OPENCV_HIGHGUI = opencv_highgui340
OPENCV_IMGPROC = opencv_imgproc340
OPENCV_VIDEO = opencv_video340
OPENCV_VIDEOIO = opencv_videoio340
}
INCLUDEPATH += "c:/OneDrive/eyeblink_libraries_msvc2017/opencv-3.4/build_release_enabledebug/install/include"
LIBS += -L"c:/OneDrive/eyeblink_libraries_msvc2017/opencv-3.4/build_release_enabledebug/install/x64/vc15/lib"
LIBS += -L"c:/OneDrive/eyeblink_libraries_msvc2017/opencv-3.4/build_release_enabledebug/install/x64/vc15/bin" -l$$OPENCV_CORE -l$$OPENCV_HIGHGUI -l$$OPENCV_IMGPROC -l$$OPENCV_VIDEO -l$$OPENCV_VIDEOIO
---------------
#include <QCoreApplication>
#include "opencv2/opencv.hpp"
#include <iostream>
int main(int argc, char *argv[])
{
cv::VideoCapture cam;
cam.open(0);
cv::Mat image;
for(int i=0;i<100;i++)
{
cam>> image;
std::cout<<i<<" ";
}
cam.release();
return 0;
}
| category: videoio(camera),incomplete | low | Critical |
305,559,592 | angular | Add ability to provide reason to navigation cancellation. | ## I'm submitting a...
<pre><code>
[ ] Regression (a behavior that used to work and stopped working in a new release)
[ ] Bug report
[ x ] Feature request
[ ] Documentation issue or request
[ ] Support request
</code></pre>
## Current behavior
When blocking route resolution via a route guard, the public API permits returning a boolean. In the router, this is then mapped to a `NavigationCancel` event with an empty message. As there are many different reasons why a route may be cancelled ("Insufficient access rights", "You're not logged in", "You're logged in", for instance...), it would be nice if that reason can be provided when cancelling a route.
The hooks for this already exist. If an error is thrown, that matches the `isNavigationCancellingError` evaluation, then a message is attached to the subsequent `NavigationCancel` event. However, the `navigationCancellingError` method that builds these errors is not exposed via the module bundle.
## Expected behavior
I should be able to provide a reason for cancelling navigation, via a route guard. This can either be via a NavigationCancellingError, or by providing an additional type of result that may be returned from methods like `canActivate`.
## What is the motivation / use case for changing the behavior?
Strong permission control at the route level.
## Environment
<pre><code>
Angular version: 5.2.6
</pre></code>
Browser:
- [ latest ] Chrome (desktop)
For Tooling issues:
- Node version: 8.9.1
- Platform: Mac
Others:
<!-- Anything else relevant? Operating system version, IDE, package manager, HTTP server, ... -->
</code></pre>
| feature,freq2: medium,area: router,feature: under consideration | medium | Critical |
305,637,373 | flutter | Please clarify the usage of InheritedWidget | Hi,
after long discussions in Gitter today I hope you can shed some light on that topic.
What is the correct way to use an InheritedWidget? So far I understood that it gives you the chance to propagate data down the Widget tree. In extreme if you put is as RootWidget it will be accessible from all Widgets in the tree on all Routes, which is fine because somehow I have to make my ViewModel/Model accessible for my Widgets without having to resort to globals or Singletons.
BUT InheritedWidget is immutable, so how can I update it? And more important how are my Stateful Widgets triggered to rebuild their subtrees?
Unfortunately the documentation is here very unclear and after discussion with a lot of people including @brianegan nobody seems really to know what the correct way of using it.
Thanks a lot for your great work! I really like Flutter coming from Xamarin!
Thomas | framework,d: api docs,c: proposal,P2,team-framework,triaged-framework | low | Major |
305,644,066 | flutter | flutter run's "o" switch-operating-system command needs a 'reset' to default option | When using
```bash
flutter run -d
```
to enable hot-reloading on an iOS & Android emulator at the same time... when a user then presses "o"
```bash
To simulate different operating systems, (defaultTargetPlatform), press "o".
```
to switch operating systems, both change to iOS, then to Android, then back to 'iOS', and so on if you keep pressing "o".
Perhaps an option needs to be added (eg "O") to reset them to their original operating system?
Of course you can just do "R" to do a full restart but then you lose state.
(Tiny non important issue, but thought I'd log it anyway)
| c: new feature,tool,P3,team-tool,triaged-tool | low | Minor |
305,666,718 | TypeScript | Proposal: better type narrowing in overloaded functions through overload set pruning | **TypeScript Version:** 2.7.2
**Search Terms:** type inference, type narrowing, overloads
**Code**
```ts
function bar(selector: "A", val: number): number;
function bar(selector: "B", val: string): string;
function bar(selector: "A" | "B", val: number | string): number | string
{
if (selector === "A")
{
const the_selector = selector; // "A"
const the_val = val; // number | string but should be just number
return "foo"; // should give error: when selector is "A" should return a number
}
else
{
const the_selector = selector; // "B"
const the_val = val; // number | string but should be just string
return 42; // should give error: when selector is "B" should return a string
}
}
```
**Issue**
The snippet above shows a limitation of the current type inference implementation in TypeScript.
As can be clearly seen from the overloaded declarations, when `selector === "A"` we have `val: number` and we must return a `number`, while when `selector === "B"` we have `val: string` and we must return a `string`. But the type-checker, although able to understand that `the_selector` can only be `"A"` in the `if` block and only `"B"` in the `else` block, still types `the_val` as `number | string` in both blocks and allows us to return a `string` when `selector === "A"` and a `number` when `selector === "B"`.
**Possible Solution**
In the implementation of an overloaded function, when the type-checker decides to narrow the type of a parameter (as often happens in `if-else` blocks), it should also remove the overloads that don't match the narrowed parameter type from the overload set, and use the pruned overload set to narrow the types of the other parameters.
**Playground Link:** https://www.typescriptlang.org/play/#src=%0Afunction%20bar(selector%3A%20%22A%22%2C%20val%3A%20number)%3A%20number%3B%0Afunction%20bar(selector%3A%20%22B%22%2C%20val%3A%20string)%3A%20string%3B%0Afunction%20bar(selector%3A%20%22A%22%20%7C%20%22B%22%2C%20val%3A%20number%20%7C%20string)%3A%20number%20%7C%20string%0A%7B%0A%20%20%20%20if%20(selector%20%3D%3D%3D%20%22A%22)%0A%20%20%20%20%7B%0A%20%20%20%20%20%20%20%20const%20the_selector%20%3D%20selector%3B%0A%20%20%20%20%20%20%20%20const%20the_val%20%3D%20val%3B%0A%20%20%20%20%20%20%20%20return%20%22foo%22%3B%0A%20%20%20%20%7D%0A%20%20%20%20else%0A%20%20%20%20%7B%0A%20%20%20%20%20%20%20%20const%20the_selector%20%3D%20selector%3B%0A%20%20%20%20%20%20%20%20const%20the_val%20%3D%20val%3B%0A%20%20%20%20%20%20%20%20return%2042%3B%0A%20%20%20%20%7D%0A%7D
| Suggestion,In Discussion | medium | Critical |
305,672,802 | youtube-dl | ProjectAlpha Support / Support for Comcast's theplatform.com | ### Make sure you are using the *latest* version: run `youtube-dl --version` and ensure your version is *2018.03.14*. If it's not, read [this FAQ entry](https://github.com/rg3/youtube-dl/blob/master/README.md#how-do-i-update-youtube-dl) and update. Issues with outdated version will be rejected.
- [X] I've **verified** and **I assure** that I'm running youtube-dl **2018.03.14**
### Before submitting an *issue* make sure you have:
- [X] At least skimmed through the [README](https://github.com/rg3/youtube-dl/blob/master/README.md), **most notably** the [FAQ](https://github.com/rg3/youtube-dl#faq) and [BUGS](https://github.com/rg3/youtube-dl#bugs) sections
- [X] [Searched](https://github.com/rg3/youtube-dl/search?type=Issues) the bugtracker for similar issues including closed ones
- [X] Checked that provided video/audio/playlist URLs (if any) are alive and playable in a browser
### What is the purpose of your *issue*?
- [X] Bug report (encountered problems with youtube-dl)
- [X] Site support request (request for adding support for a new site)
- [ ] Feature request (request for a new functionality)
- [ ] Question
- [ ] Other
---
I've recently attempted to use youtube-dl with content hosted on Legendary Entertainment's projectalpha.com to no avail. A great deal of their content is available on VRV's premium option, but there is some Alpha-exclusive content. I was wanting to archive some older shows into Plex, but my attempts so far have failed.
When trying to run youtube-dl against the domain in the browser I get a message regarding it being an unsupported site - however the site levies a streaming platform developed by Comcast, so it's not just specific to them. For a test video I was able to snag the link to a .m3u8 file for a specific quality video using a Chrome extension, [Video DownloadHelper](https://chrome.google.com/webstore/detail/video-downloadhelper/lmjnegcaeklhafolokijcfjliaokphfk?hl=en-US).
---
### youtube-dl ran against an episode on projectalpha.com
```
youtube-dl.exe https://www.projectalpha.com/browse/#svod?method=video&videoId=GaR5mX94hxr57MUJ_v_zyGJ1A_U4GqwH -v
[generic] #svod?method=video: Requesting header
WARNING: Falling back on generic information extractor.
[generic] #svod?method=video: Downloading webpage
[generic] #svod?method=video: Extracting information
ERROR: Unsupported URL: https://www.projectalpha.com/browse/#svod?method=video
'videoId' is not recognized as an internal or external command,
operable program or batch file.
```
---
### youtube-dl ran against the .m3u8 file
```
youtube-dl.exe https://edge.legendary.top.comcast.net/default/Legendary/333/428/1521058040591/20180313_g_talksmachina_2009_2997_Alpha20_23971909671_m3u8_video_1920x1080_8000000_primary_audio_eng_xc22975e5765a419e85b930d3b899b6e9_8.m3u8 -v
[debug] System config: []
[debug] User config: []
[debug] Custom config: []
[debug] Command-line args: ['https://edge.legendary.top.comcast.net/default/Legendary/333/428/1521058040591/20180313_g_talksmachina_2009_2997_Alpha20_23971909671_m3u8_video_1920x1080_8000000_primary_audio_eng_xc22975e5765a419e85b930d3b899b6e9_8.m3u8', '-v']
[debug] Encodings: locale cp1252, fs mbcs, out cp437, pref cp1252
[debug] youtube-dl version 2018.03.14
[debug] Python version 3.4.4 (CPython) - Windows-10-10.0.16299
[debug] exe versions: ffmpeg N-90315-gf706cdda56, ffprobe N-90315-gf706cdda56
[debug] Proxy map: {}
[generic] 20180313_g_talksmachina_2009_2997_Alpha20_23971909671_m3u8_video_1920x1080_8000000_primary_audio_eng_xc22975e5765a419e85b930d3b899b6e9_8: Requesting header
[generic] 20180313_g_talksmachina_2009_2997_Alpha20_23971909671_m3u8_video_1920x1080_8000000_primary_audio_eng_xc22975e5765a419e85b930d3b899b6e9_8: Downloading m3u8 information
[debug] Default format spec: bestvideo+bestaudio/best
[debug] Invoking downloader on 'https://edge.legendary.top.comcast.net/default/Legendary/333/428/1521058040591/20180313_g_talksmachina_2009_2997_Alpha20_23971909671_m3u8_video_1920x1080_8000000_primary_audio_eng_xc22975e5765a419e85b930d3b899b6e9_8.m3u8'
[download] Destination: 20180313_g_talksmachina_2009_2997_Alpha20_23971909671_m3u8_video_1920x1080_8000000_primary_audio_eng_xc22975e5765a419e85b930d3b899b6e9_8-20180313_g_talksmachina_2009_2997_Alpha20_23971909671_m3u8_video_1920x1080_8000000_primary_audio_eng_xc22975e5765a419e85b930d3b899b6e9_8.mp4
[debug] ffmpeg command line: ffmpeg -y -loglevel verbose -headers "Accept-Encoding: gzip, deflate
Accept-Charset: ISO-8859-1,utf-8;q=0.7,*;q=0.7
Accept: text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8
User-Agent: Mozilla/5.0 (X11; Linux x86_64; rv:59.0) Gecko/20100101 Firefox/59.0 (Chrome)
Accept-Language: en-us,en;q=0.5
" -i "https://edge.legendary.top.comcast.net/default/Legendary/333/428/1521058040591/20180313_g_talksmachina_2009_2997_Alpha20_23971909671_m3u8_video_1920x1080_8000000_primary_audio_eng_xc22975e5765a419e85b930d3b899b6e9_8.m3u8" -c copy -f mp4 "-bsf:a" aac_adtstoasc "file:20180313_g_talksmachina_2009_2997_Alpha20_23971909671_m3u8_video_1920x1080_8000000_primary_audio_eng_xc22975e5765a419e85b930d3b899b6e9_8-20180313_g_talksmachina_2009_2997_Alpha20_23971909671_m3u8_video_1920x1080_8000000_primary_audio_eng_xc22975e5765a419e85b930d3b899b6e9_8.mp4.part"
ffmpeg version N-90315-gf706cdda56 Copyright (c) 2000-2018 the FFmpeg developers
built with gcc 7.3.0 (GCC)
configuration: --enable-gpl --enable-version3 --enable-sdl2 --enable-bzlib --enable-fontconfig --enable-gnutls --enable-iconv --enable-libass --enable-libbluray --enable-libfreetype --enable-libmp3lame --enable-libopencore-amrnb --enable-libopencore-amrwb --enable-libopenjpeg --enable-libopus --enable-libshine --enable-libsnappy --enable-libsoxr --enable-libtheora --enable-libtwolame --enable-libvpx --enable-libwavpack --enable-libwebp --enable-libx264 --enable-libx265 --enable-libxml2 --enable-libzimg --enable-lzma --enable-zlib --enable-gmp --enable-libvidstab --enable-libvorbis --enable-libvo-amrwbenc --enable-libmysofa --enable-libspeex --enable-libxvid --enable-libmfx --enable-amf --enable-ffnvcodec --enable-cuvid --enable-d3d11va --enable-nvenc --enable-nvdec --enable-dxva2 --enable-avisynth
libavutil 56. 9.100 / 56. 9.100
libavcodec 58. 14.100 / 58. 14.100
libavformat 58. 10.100 / 58. 10.100
libavdevice 58. 2.100 / 58. 2.100
libavfilter 7. 13.100 / 7. 13.100
libswscale 5. 0.102 / 5. 0.102
libswresample 3. 0.101 / 3. 0.101
libpostproc 55. 0.100 / 55. 0.100
[hls,applehttp @ 000001b453e7ae00] HLS request for url 'https://edge.legendary.top.comcast.net/default/Legendary/333/428/1521058040591/20180313_g_talksmachina_2009_2997_Alpha20_23971909671_m3u8_video_1920x1080_8000000_primary_audio_eng_8_xc22975e5765a419e85b930d3b899b6e9_00001.ts', offset 0, playlist 0
[hls,applehttp @ 000001b453e7ae00] Opening 'https://edge.legendary.top.comcast.net/default/Legendary/333/428/1521058040591/20180313_g_talksmachina_2009_2997_Alpha20_23971909671_m3u8_video_1920x1080_8000000_primary_audio_eng_8_xc22975e5765a419e85b930d3b899b6e9_00001.ts' for reading
[hls,applehttp @ 000001b453e7ae00] HLS request for url 'https://edge.legendary.top.comcast.net/default/Legendary/333/428/1521058040591/20180313_g_talksmachina_2009_2997_Alpha20_23971909671_m3u8_video_1920x1080_8000000_primary_audio_eng_8_xc22975e5765a419e85b930d3b899b6e9_00002.ts', offset 0, playlist 0
[hls,applehttp @ 000001b453e7ae00] Opening 'https://edge.legendary.top.comcast.net/default/Legendary/333/428/1521058040591/20180313_g_talksmachina_2009_2997_Alpha20_23971909671_m3u8_video_1920x1080_8000000_primary_audio_eng_8_xc22975e5765a419e85b930d3b899b6e9_00002.ts' for reading
[mpegts @ 000001b455ca2180] parser not found for codec timed_id3, packets or times may be invalid.
[h264 @ 000001b456124e40] Reinit context to 1920x1088, pix_fmt: yuv420p
Input #0, hls,applehttp, from 'https://edge.legendary.top.comcast.net/default/Legendary/333/428/1521058040591/20180313_g_talksmachina_2009_2997_Alpha20_23971909671_m3u8_video_1920x1080_8000000_primary_audio_eng_xc22975e5765a419e85b930d3b899b6e9_8.m3u8':
Duration: 01:22:42.42, start: 2.059589, bitrate: 0 kb/s
Program 0
Metadata:
variant_bitrate : 0
Stream #0:0: Video: h264 (High), 1 reference frame ([27][0][0][0] / 0x001B), yuv420p(left), 1920x1080 (1920x1088) [SAR 1:1 DAR 16:9], 29.97 fps, 29.97 tbr, 90k tbn, 59.94 tbc
Metadata:
variant_bitrate : 0
Stream #0:1: Audio: aac (LC) ([15][0][0][0] / 0x000F), 44100 Hz, stereo, fltp
Metadata:
variant_bitrate : 0
Stream #0:2: Data: timed_id3 (ID3 / 0x20334449)
Metadata:
variant_bitrate : 0
file:20180313_g_talksmachina_2009_2997_Alpha20_23971909671_m3u8_video_1920x1080_8000000_primary_audio_eng_xc22975e5765a419e85b930d3b899b6e9_8-20180313_g_talksmachina_2009_2997_Alpha20_23971909671_m3u8_video_1920x1080_8000000_primary_audio_eng_xc22975e5765a419e85b930d3b899b6e9_8.mp4.part: No such file or directory
ERROR: ffmpeg exited with code 1
File "__main__.py", line 19, in <module>
File "C:\Users\dst\AppData\Roaming\Build archive\youtube-dl\rg3\tmph71axfm2\build\youtube_dl\__init__.py", line 471, in main
File "C:\Users\dst\AppData\Roaming\Build archive\youtube-dl\rg3\tmph71axfm2\build\youtube_dl\__init__.py", line 461, in _real_main
File "C:\Users\dst\AppData\Roaming\Build archive\youtube-dl\rg3\tmph71axfm2\build\youtube_dl\YoutubeDL.py", line 1989, in download
File "C:\Users\dst\AppData\Roaming\Build archive\youtube-dl\rg3\tmph71axfm2\build\youtube_dl\YoutubeDL.py", line 796, in extract_info
File "C:\Users\dst\AppData\Roaming\Build archive\youtube-dl\rg3\tmph71axfm2\build\youtube_dl\YoutubeDL.py", line 850, in process_ie_result
File "C:\Users\dst\AppData\Roaming\Build archive\youtube-dl\rg3\tmph71axfm2\build\youtube_dl\YoutubeDL.py", line 1623, in process_video_result
File "C:\Users\dst\AppData\Roaming\Build archive\youtube-dl\rg3\tmph71axfm2\build\youtube_dl\YoutubeDL.py", line 1896, in process_info
File "C:\Users\dst\AppData\Roaming\Build archive\youtube-dl\rg3\tmph71axfm2\build\youtube_dl\YoutubeDL.py", line 1835, in dl
File "C:\Users\dst\AppData\Roaming\Build archive\youtube-dl\rg3\tmph71axfm2\build\youtube_dl\downloader\common.py", line 364, in download
File "C:\Users\dst\AppData\Roaming\Build archive\youtube-dl\rg3\tmph71axfm2\build\youtube_dl\downloader\external.py", line 57, in real_download
File "C:\Users\dst\AppData\Roaming\Build archive\youtube-dl\rg3\tmph71axfm2\build\youtube_dl\downloader\common.py", line 166, in report_error
File "C:\Users\dst\AppData\Roaming\Build archive\youtube-dl\rg3\tmph71axfm2\build\youtube_dl\YoutubeDL.py", line 613, in report_error
File "C:\Users\dst\AppData\Roaming\Build archive\youtube-dl\rg3\tmph71axfm2\build\youtube_dl\YoutubeDL.py", line 575, in trouble
```
---
### Account required
An account is required to access content on Alpha - I can provide one if necessary. There's also a 60 day free promo code right now (QUESTION).
https://twitter.com/Marisha_Ray/status/971820448596951040 | account-needed | low | Critical |
305,686,985 | vue | keep-alive breaks initial transition | ### Version
2.5.16 (but I also checked to version 2.5.5)
### Reproduction link
[https://jsfiddle.net/bpkpz6v6/](https://jsfiddle.net/bpkpz6v6/)
### Steps to reproduce
- Add 3 items. You can see the animation on every item ✅
- Remove items in this order 3 -> 2 -> 1
- Add 3 item. This time animation for item 1 is not being applied ❌
- Remove items but in reverse order 1 -> 2 -> 3
- Again add items. Animation works correctly again ✅
### What is expected?
Transition to work every time
### What is actually happening?
When transition-group inside keep-alive is activated after being deactivated, it sometimes does't apply a transition on the first rendered element.
<!-- generated by vue-issues. DO NOT REMOVE --> | transition | medium | Minor |
305,696,975 | godot | Light2D misbehaves if nodes use custom draw() | Godot 3.02
Windows 7, 64bit
Nvidia Geforce GTX 960
**Issue description:**
Using custom draw() commands with nodes does not work properly with Light2D if drawn areas overlap.
Lighting seems to accumulate at the overlapping areas.
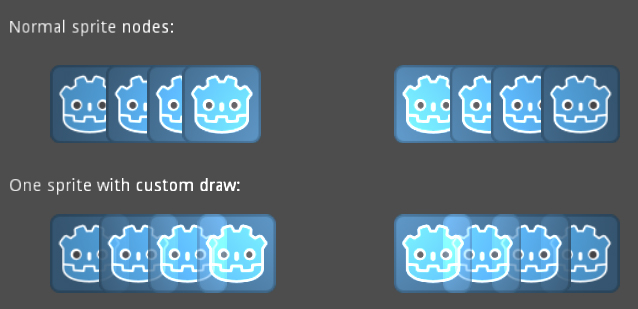
**Steps to reproduce:**
Create any CanvasItem that uses custom draw commands that create overlapping graphics. Add a Light2D.
**Minimal reproduction project:**
[light2d_custom_draw.zip](https://github.com/godotengine/godot/files/1816889/light2d_custom_draw.zip)
| bug,topic:rendering,confirmed,topic:2d | low | Major |
305,719,676 | go | cmd/compile: optimize large structs | The compiler currently compiles large structs conservatively. If a struct type has more than 4 fields (or a few other conditions), we treat that type as unSSAable. All operations on variables of that type go to the stack, as if their address was taken.
This is suboptimal in various ways. For example:
```
type T struct {
a, b, c, d int
}
func f(x *T) {
t := T{}
*x = t
}
type U struct {
a, b, c, d, e int
}
func g(x *U) {
u := U{}
*x = u
}
```
`f` is compiled optimally, to:
```
XORPS X0, X0
MOVQ "".x+8(SP), AX
MOVUPS X0, (AX)
MOVUPS X0, 16(AX)
RET
```
`g` is quite a bit worse:
``` SUBQ $48, SP
MOVQ BP, 40(SP)
LEAQ 40(SP), BP
MOVQ $0, "".u(SP)
XORPS X0, X0
MOVUPS X0, "".u+8(SP)
MOVUPS X0, "".u+24(SP)
MOVQ "".u(SP), AX
MOVQ "".x+56(SP), CX
MOVQ AX, (CX)
LEAQ 8(CX), DI
LEAQ "".u+8(SP), SI
DUFFCOPY $868
MOVQ 40(SP), BP
ADDQ $48, SP
RET
```
We zero a temporary variable on the stack, then copy it to the destination.
We should process large structs through SSA as well. This will require a fair amount of work in the SSA backend to introduce struct builders, selectors of arbitrary width, stack allocation of large types, maybe heap allocation if they are really huge, etc.
Arrays of size > 1 are in a similar state, but they are somewhat harder because non-constant indexes add an additional complication.
| Performance,NeedsFix,compiler/runtime | medium | Major |
305,720,628 | go | x/build/cmd/gopherbot: comment patch series in order | When I upload a patch series, it would be nice if those CLs were grouped together as a single gopherbot comment on the issue, or at least listed in CL order.
Here's an example where gopherbot listed CL 100846 before CL 100845: https://github.com/golang/go/issues/22075#issuecomment-373522789 | Builders,NeedsFix | low | Minor |
305,723,167 | rust | rayon type inference regression | The rayon tests are encountering a new type inference failure, and with the latest nightly, an ICE.
```
$ git describe HEAD
v1.0.0-14-g68aabe9e460f
$ rustc +nightly-2018-03-15 -Vv
rustc 1.26.0-nightly (521d91c6b 2018-03-14)
binary: rustc
commit-hash: 521d91c6be76367d966df419677dd187f799b116
commit-date: 2018-03-14
host: x86_64-unknown-linux-gnu
release: 1.26.0-nightly
LLVM version: 6.0
$ cargo +nightly-2018-03-15 test --lib --no-run
Compiling [deps...]
Compiling rayon v1.0.0 (file:///home/jistone/rust/rayon)
error[E0283]: type annotations required: cannot resolve `_: std::iter::Sum<&usize>`
--> src/iter/test.rs:283:54
|
283 | assert_eq!(num.load(Ordering::Relaxed), a.iter().sum());
| ^^^
error: internal compiler error: librustc/ich/impls_ty.rs:907: ty::TypeVariants::hash_stable() - Unexpected variant TyInfer(?0).
thread 'rustc' panicked at 'Box<Any>', librustc_errors/lib.rs:540:9
note: Some details are omitted, run with `RUST_BACKTRACE=full` for a verbose backtrace.
stack backtrace:
0: std::sys::unix::backtrace::tracing::imp::unwind_backtrace
at libstd/sys/unix/backtrace/tracing/gcc_s.rs:49
1: std::sys_common::backtrace::_print
at libstd/sys_common/backtrace.rs:71
2: std::panicking::default_hook::{{closure}}
at libstd/sys_common/backtrace.rs:59
at libstd/panicking.rs:207
3: std::panicking::default_hook
at libstd/panicking.rs:223
4: core::ops::function::Fn::call
5: std::panicking::rust_panic_with_hook
at libstd/panicking.rs:403
6: std::panicking::begin_panic
7: rustc_errors::Handler::bug
8: rustc::session::opt_span_bug_fmt::{{closure}}
9: rustc::ty::context::tls::with_opt::{{closure}}
10: <std::thread::local::LocalKey<T>>::try_with
11: <std::thread::local::LocalKey<T>>::with
12: rustc::ty::context::tls::with
13: rustc::ty::context::tls::with_opt
14: rustc::session::opt_span_bug_fmt
15: rustc::session::bug_fmt
16: rustc::ich::impls_ty::<impl rustc_data_structures::stable_hasher::HashStable<rustc::ich::hcx::StableHashingContext<'a>> for rustc::ty::sty::TypeVariants<'gcx>>::hash_stable
17: rustc::dep_graph::dep_node::DepNode::new
18: rustc::ty::maps::<impl rustc::ty::maps::queries::dropck_outlives<'tcx>>::try_get
19: rustc::ty::maps::TyCtxtAt::dropck_outlives
20: rustc::traits::query::dropck_outlives::<impl rustc::infer::at::At<'cx, 'gcx, 'tcx>>::dropck_outlives
21: rustc_typeck::check::dropck::check_safety_of_destructor_if_necessary
22: rustc::hir::Pat::walk_
23: <rustc_typeck::check::regionck::RegionCtxt<'a, 'gcx, 'tcx> as rustc::hir::intravisit::Visitor<'gcx>>::visit_local
24: rustc::hir::intravisit::walk_expr
25: <rustc_typeck::check::regionck::RegionCtxt<'a, 'gcx, 'tcx> as rustc::hir::intravisit::Visitor<'gcx>>::visit_expr
26: rustc_typeck::check::regionck::RegionCtxt::visit_fn_body
27: rustc_typeck::check::typeck_tables_of::{{closure}}
28: rustc_typeck::check::typeck_tables_of
29: rustc::dep_graph::graph::DepGraph::with_task_impl
30: rustc::ty::maps::<impl rustc::ty::maps::queries::typeck_tables_of<'tcx>>::force
31: rustc::ty::maps::<impl rustc::ty::maps::queries::typeck_tables_of<'tcx>>::try_get
32: rustc::ty::maps::TyCtxtAt::typeck_tables_of
33: rustc::ty::maps::<impl rustc::ty::maps::queries::typeck_tables_of<'tcx>>::ensure
34: rustc_typeck::check::typeck_item_bodies
35: rustc::dep_graph::graph::DepGraph::with_task_impl
36: rustc::ty::maps::<impl rustc::ty::maps::queries::typeck_item_bodies<'tcx>>::force
37: rustc::ty::maps::<impl rustc::ty::maps::queries::typeck_item_bodies<'tcx>>::try_get
38: rustc::ty::maps::TyCtxtAt::typeck_item_bodies
39: rustc::ty::maps::<impl rustc::ty::context::TyCtxt<'a, 'tcx, 'lcx>>::typeck_item_bodies
40: rustc_typeck::check_crate
41: rustc::ty::context::TyCtxt::create_and_enter
42: rustc_driver::driver::compile_input
43: rustc_driver::run_compiler
note: the compiler unexpectedly panicked. this is a bug.
note: we would appreciate a bug report: https://github.com/rust-lang/rust/blob/master/CONTRIBUTING.md#bug-reports
note: rustc 1.26.0-nightly (521d91c6b 2018-03-14) running on x86_64-unknown-linux-gnu
note: compiler flags: -C debuginfo=2 -C incremental
note: some of the compiler flags provided by cargo are hidden
error: Could not compile `rayon`.
```
With nightly-2018-03-07, it reports the same "type annotations required" error, but no ICE.
The error itself is new to us, as just a few days ago we had no problem in CI. I narrowed this down to an update to `compilertest_rs`, from 0.3.7 to 0.3.8, even though that crate is not directly involved in the test that has the error. With 0.3.7, even the currently nightly is fine. With 0.3.8, nightly-2018-03-15 has the error and ICE, and nightly-2018-03-07 back to 2018-01-28 just have the error. (Earlier than that, 0.3.8 doesn't compile at all due to differences in the `test` crate.) | P-medium,T-compiler,A-inference,C-bug,E-needs-mcve | low | Critical |
305,734,440 | rust | When running Emscripten tests, rustbuild should tell compilertest the version of the Emscripten LLVM, not the host LLVM | Discovered in https://github.com/rust-lang/rust/pull/48983#issuecomment-373529195. It seems rustbuild will always passes information about the host LLVM config, even when running Emscripten tests. https://github.com/rust-lang/rust/blob/39264539448e7ec5e98067859db71685393a4464/src/bootstrap/test.rs#L911-L920
This makes `min-llvm-version` reports the wrong value and failed to ignore some tests.
cc #48757 (probably a regression introduced by this PR) | A-LLVM,A-testsuite,A-cross,T-bootstrap,C-bug,O-emscripten | low | Critical |
305,746,566 | TypeScript | Infer type in conditional cannot unify generics | <!-- 🚨 STOP 🚨 𝗦𝗧𝗢𝗣 🚨 𝑺𝑻𝑶𝑷 🚨 -->
<!--
Half of all issues filed here are duplicates, answered in the FAQ, or not appropriate for the bug tracker.
Please help us by doing the following steps before logging an issue:
* Search: https://github.com/Microsoft/TypeScript/search?type=Issues
* Read the CONTRIBUTING guidelines: https://github.com/Microsoft/TypeScript/blob/master/CONTRIBUTING.md
* Read the FAQ: https://github.com/Microsoft/TypeScript/wiki/FAQ
-->
<!-- If you have a QUESTION:
THIS IS NOT A FORUM FOR QUESTIONS.
Ask questions at http://stackoverflow.com/questions/tagged/typescript
or https://gitter.im/Microsoft/TypeScript
-->
<!-- If you have a SUGGESTION:
Most suggestion reports are duplicates, please search extra hard before logging a new suggestion.
See https://github.com/Microsoft/TypeScript-wiki/blob/master/Writing-Good-Design-Proposals.md
-->
<!-- If you have a BUG:
Please fill in the *entire* template below.
-->
<!-- Please try to reproduce the issue with `typescript@next`. It may have already been fixed. -->
**TypeScript Version:** 2.8.0-dev.20180315
<!-- Search terms you tried before logging this (so others can find this issue more easily) -->
**Search Terms:**
infer conditional unify generic function
**Code**
```ts
type Apply1<T, V> = T extends (item: V) => infer R ? R : never;
type A1 = Apply1< (item: string) => string[] , string>;
type B1 = Apply1< <U>(item: U) => U[], string>;
type Apply2<T, V> = T extends (item: V, obj: infer R) => any ? R : never;
type A2 = Apply2< (item: string, obj: string[]) => any , string>;
type B2 = Apply2< <U>(item: U, obj: U[]) => any , string>;
type Apply3<T, V> = T extends (item: V, obj: infer R) => infer R ? R : never;
type A3 = Apply3< (item: string, obj: string[]) => string[] , string>;
type B3 = Apply3< <U>(item: U, obj: U[]) => U[] , string>;
```
```json
{
"compilerOptions": {
"allowJs": true,
"target": "es6",
"module": "commonjs",
"outDir": "dest",
"strictNullChecks": true,
"jsx": "preserve",
"strictFunctionTypes": true
}
}
```
**Expected behavior:**
All `A` and `B` types are inferred to `string[]`. For types `B`, the type parameter `U` should be unified with `string`.
**Actual behavior:**
All `B` types are inferred to `{}[]`.
**Related Issues:**
#22615 (different problem, but similar input) | Suggestion,Needs Proposal | low | Critical |
305,751,959 | TypeScript | In JS, aliasing property assignments to JS containers should work | ```ts
// @noEmit: true
// @allowJs: true
// @checkJs: true
// @strict: true
// @Filename: a.js
// from async
var a = {};
a.each = function () {
console.log('hi')
}
a.forEach = a.each
```
**Expected behavior:**
No error, and `a.each()` and `a.forEach()` both have the type `() => void`.
**Actual behavior:**
a, a.each and a.forEach are all of type any.
With no implicitAny on, there are two errors:
Two errors:
1. 'a' implicitly has type any because it does not have a type annotation is referenced directly or indirectly in its own intializer.
1. 'forEach' implicitly has type any because it does not have a type annotation is referenced directly or indirectly in its own intializer. | Bug,Domain: JavaScript | low | Critical |
305,850,431 | opencv | Bug(s) in cv::fitEllipse() | ##### System information (version)
- OpenCV => 3.4
- Operating System / Platform => Windows 10 64bit
- Compiler => Visual Studio 2015
##### Detailed description
#### This issue affects _**cv::fitEllipse**_ in _**shapedescr.cpp**_.
1. The angle of ellipses with a small difference in width and height (circle-like) are sometimes not assigned to the output-cv::RotatedRect.
2. Furthermore there is an incorrect approximation.
3. Some other lines appear odd to me.
##### Steps to reproduce
First of all: I am no professional and this is my first bug report. Sorry for poor formatting etc.
1. Jumping to the last lines of the function definition: The assignment of the angle value is hidden behind an if-statement which is sometimes not true. Due to this, the default value of 0 is returned with the cv::RotatedRect. The following code snippet shows a simple solution to that.
```
box.angle = (float)(rp[4] * 180 / CV_PI); // Assignment of angle moved out of if-bracket
if (box.size.width > box.size.height)
{
float tmp;
CV_SWAP(box.size.width, box.size.height, tmp);
box.angle += 90.0; // In case w and h swap - 90° should be added
//box.angle = (float)(90 + rp[4] * 180 / CV_PI);
}
```
2. The incorrect approximation is found in line 380.
` if( fabs(gfp[2]) > min_eps )`
It is not sufficient to check only `gfp[2]`. Instead it should be the whole fraction of `gfp[2] / gfp[1] - gfp[0]`.
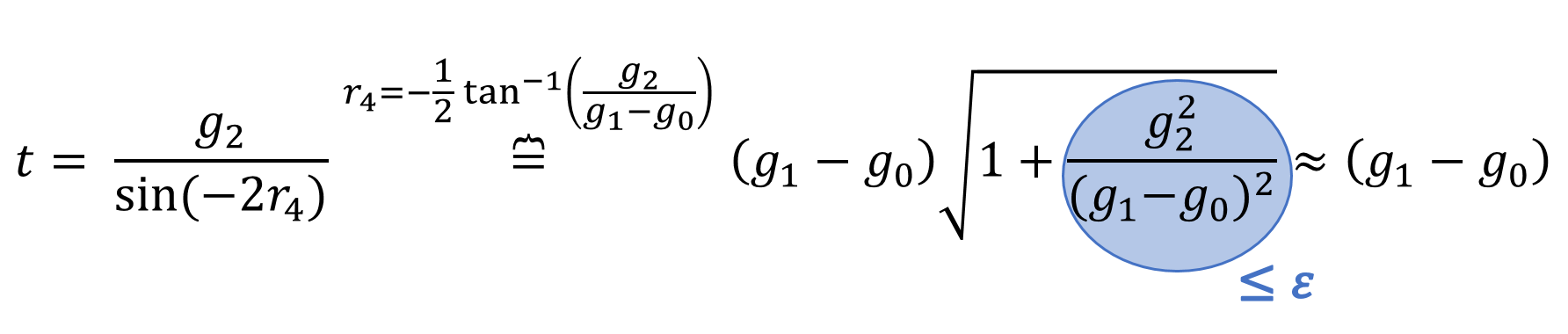
Where `g0 = gfp[0], r4 = rp[4]` and so on. The corrected line should look something like this:
`if (fabs(gfp[2]) / fabs(gfp[1] - gfp[0]) > min_eps)`
3. I do not completely understand the applied algorithm in this method but there seem to be two other odd approximations in lines 384 to 389
```
rp[2] = fabs(gfp[0] + gfp[1] - t);
if (rp[2] > min_eps) // <-- weird approximation?
rp[2] = std::sqrt(2.0 / rp[2]);
rp[3] = fabs(gfp[0] + gfp[1] + t);
if (rp[3] > min_eps) // <-- weird approximation?
rp[3] = std::sqrt(2.0 / rp[3]);
```
If you turn them around, it basically says: If width `(2*rp[2])` and height `(2*rp[3])` are small enough, they can be approximated by the square of their reciprocal value (times 4). In mathematical terms it's something like this:
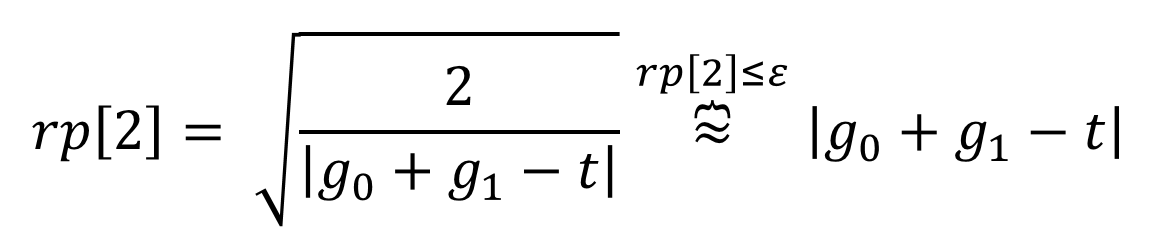
Where `g0 := gfp[0]` and `g1 := gfp[1]`. With `min_eps = 1-e8` as it is hard coded in the function, this cannot be true for any value.
I created a short example project in VS2015 on Windows.
[main.txt](https://github.com/opencv/opencv/files/1818566/main.txt)
| bug,priority: normal,category: imgproc,affected: 3.4 | low | Critical |
305,868,554 | vscode | [icon themes] Expose API to provide a dynamic icon theme. | This is an API feature request.
We at `vscode-icons` extension support project detection which dynamically re-generates our icon manifest file including or not project specific icons. Additionally to that, we provide the ability to the user to provide its own custom icons and more.
All these features, in the end, require the editor to be reloaded, simply because the icon manifest gets loaded at the start of the editor and with no ability to trigger a reload upon icon manifest recreation.
This results in a poor UX.
If we could have an API to trigger an icon manifest reload, we can have a much smoother transition between changes.
Relating request: https://github.com/vscode-icons/vscode-icons/issues/1177
//cc @robertohuertasm @jens1o @PKief @EmmanuelBeziat @LaurentTreguier @file-icons @DavidBabel @be5invis
| feature-request,themes | medium | Major |
305,876,388 | angular | Router should help manage focus for a11y | ## I'm submitting a...
<!-- Check one of the following options with "x" -->
<pre><code>
[ ] Regression (a behavior that used to work and stopped working in a new release)
[x] Bug report
[ ] Feature request
[ ] Documentation issue or request
[ ] Support request => Please do not submit support request here, instead see https://github.com/angular/angular/blob/master/CONTRIBUTING.md#question
</code></pre>
Re-opening #6106 as suggested
## Current behavior
Only the first time you navigate to a page the autofocus attribute is respected, subsequent page navigations doesn't respect the autofocus attribute.
## Expected behavior
When navigating to a page the autofocus attribute should be respected
## Minimal reproduction of the problem with instructions
Here's a minimal reproduction. https://stackblitz.com/edit/angular-jvfn5g
Follow these steps (also available in the stackblitz)
1. First navigate to Foo
2. Observe that the textbox has focus
3. Then navigate to Bar
4. Then navigate back to Foo
5. Observe that the textbox no longer has focus
## What is the motivation / use case for changing the behavior?
It is the correct and expected behavior.
## Environment
<pre><code>
Angular version: 5.2.9
Browser:
- [x] Chrome (desktop) version 65.0.3325.146
- [ ] Chrome (Android) version XX
- [ ] Chrome (iOS) version XX
- [ ] Firefox version XX
- [ ] Safari (desktop) version XX
- [ ] Safari (iOS) version XX
- [ ] IE version XX
- [ ] Edge version XX
</code></pre>
| feature,area: router,Accessibility,feature: under consideration | low | Critical |
305,889,920 | rust | macro rules shadowing error should emit where the macro being shadowed lives | The ``macro-expanded `macro_rules!`s may not shadow existing macros`` should mention where the macro being shadowed was defined.
For example compiling `stdsimd` I get the following error:
```
error: `is_x86_feature_detected` is already in scope
--> crates/stdsimd/src/../../../stdsimd/arch/detect/arch/x86.rs:21:1
|
21 | / macro_rules! is_x86_feature_detected {
22 | | ("aes") => {
23 | | cfg!(target_feature = "aes") || $crate::arch::detect::check_for(
24 | | $crate::arch::detect::Feature::aes) };
... |
165 | | };
166 | | }
| |_^
|
= note: macro-expanded `macro_rules!`s may not shadow existing macros (see RFC 1560)
error: aborting due to previous error
```
which tells me that I am defining a macro that is already defined somewhere, but it is not telling me where. That would make this diagnostic much better.
| A-diagnostics,A-macros,T-compiler,D-terse | low | Critical |
305,980,658 | godot | Sprite3D not casting a shadow | <!-- Please search existing issues for potential duplicates before filing yours:
https://github.com/godotengine/godot/issues?q=is%3Aissue
-->
**Godot version:**
<!-- Specify commit hash if non-official. -->
3.0 stable and 2.1.4 stable
**OS/device including version:**
<!-- Specify GPU model and drivers if graphics-related. -->
Phone: Oukitel K6000 Pro
Android 6.0
GPU: ARM Mali-T720 (MP3)
Driver: Open GL ES 3.1 and Open CL 1.2
**Issue description:**
When using a Sprite3D with "alpha_cut" set to "Opaque Prepass" the drop shadow is missing on my Oukitel K6000 Pro. This happens in all versions of Godot I tested and seems to be device specific. I tested with two other phones (Samsung Galaxy S3 and Galaxy J5 Duos) and the issue only occurs on the K6000 Pro.
I am using Godot 2 for my project, so it would be glad it could be fixed there, too. But I tested and uploaded a test project for godot 3 to help fixing the issue on the current version.
**Steps to reproduce:**
1. Set up a basic project
2. Add a directional Light, a Sprite3D and some object to cast a shadow on.
3. Set the "alpha_cut" of the sprite to "Opaque Prepass"
**Minimal reproduction project:**
<!-- Recommended as it greatly speeds up debugging. Drag and drop a zip archive to upload it. -->
Godot2: [Sprite3DShadowTest_Godot2.zip](https://github.com/godotengine/godot/files/1819778/Sprite3DShadowTest_Godot2.zip)
Godot3: [Sprite3DShadowTest_Godot3.zip](https://github.com/godotengine/godot/files/1819850/Sprite3DShadowTest_Godot3.zip)
**Screenshots Godot2 Project**
Oukitel:
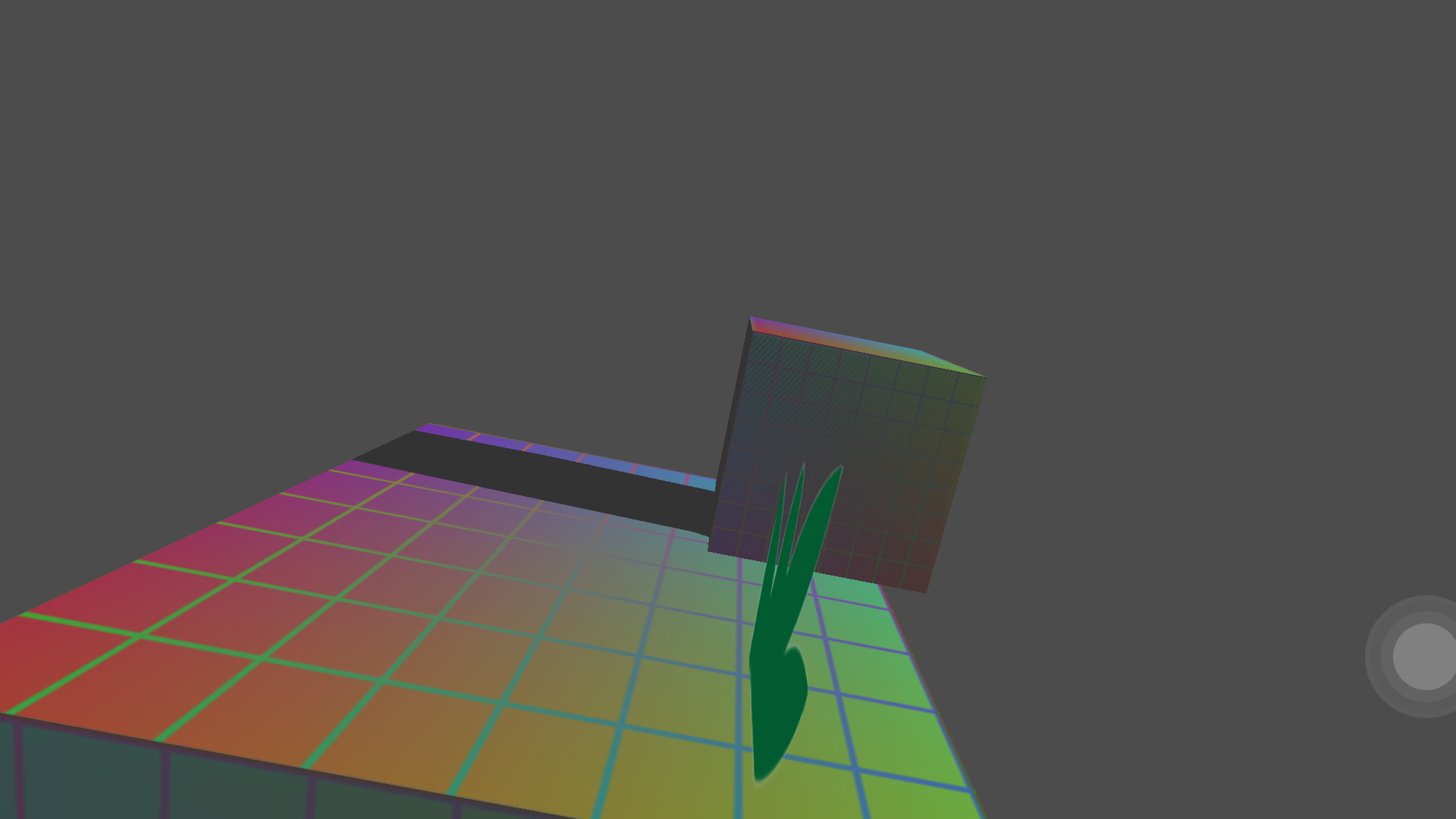
Other devices:
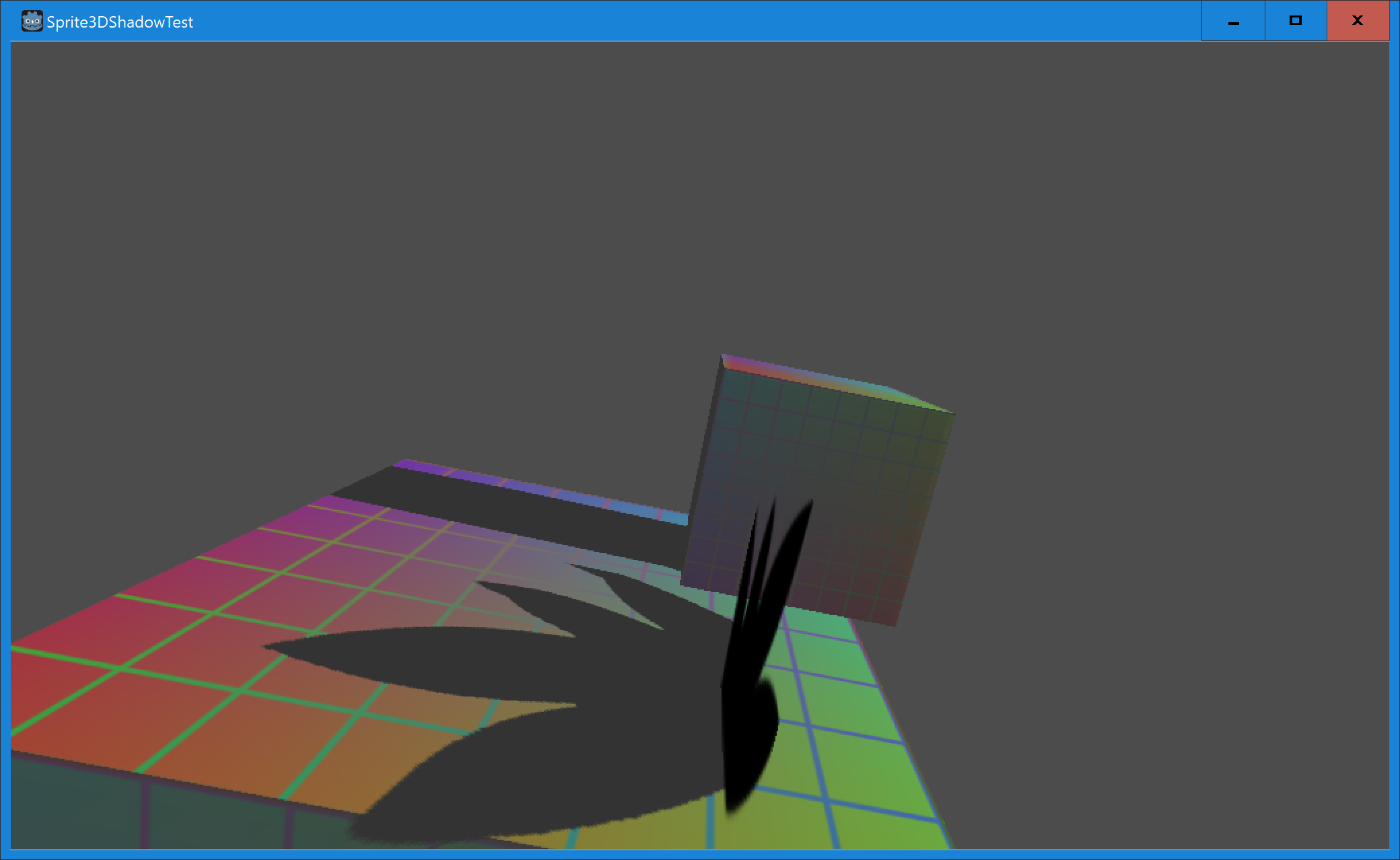
**Screenshots Godot3 Project**
Oukitel:
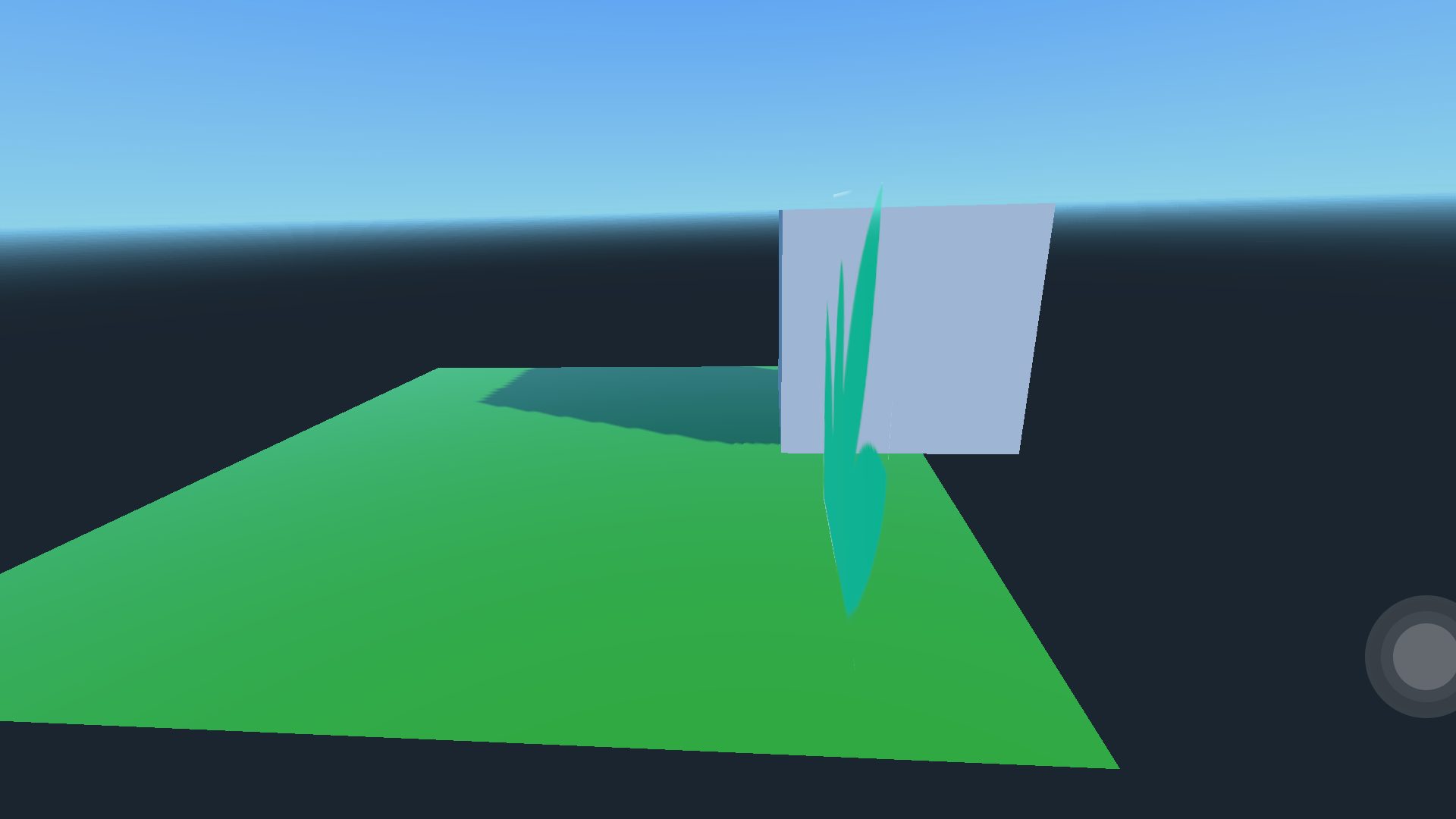
Other devices:
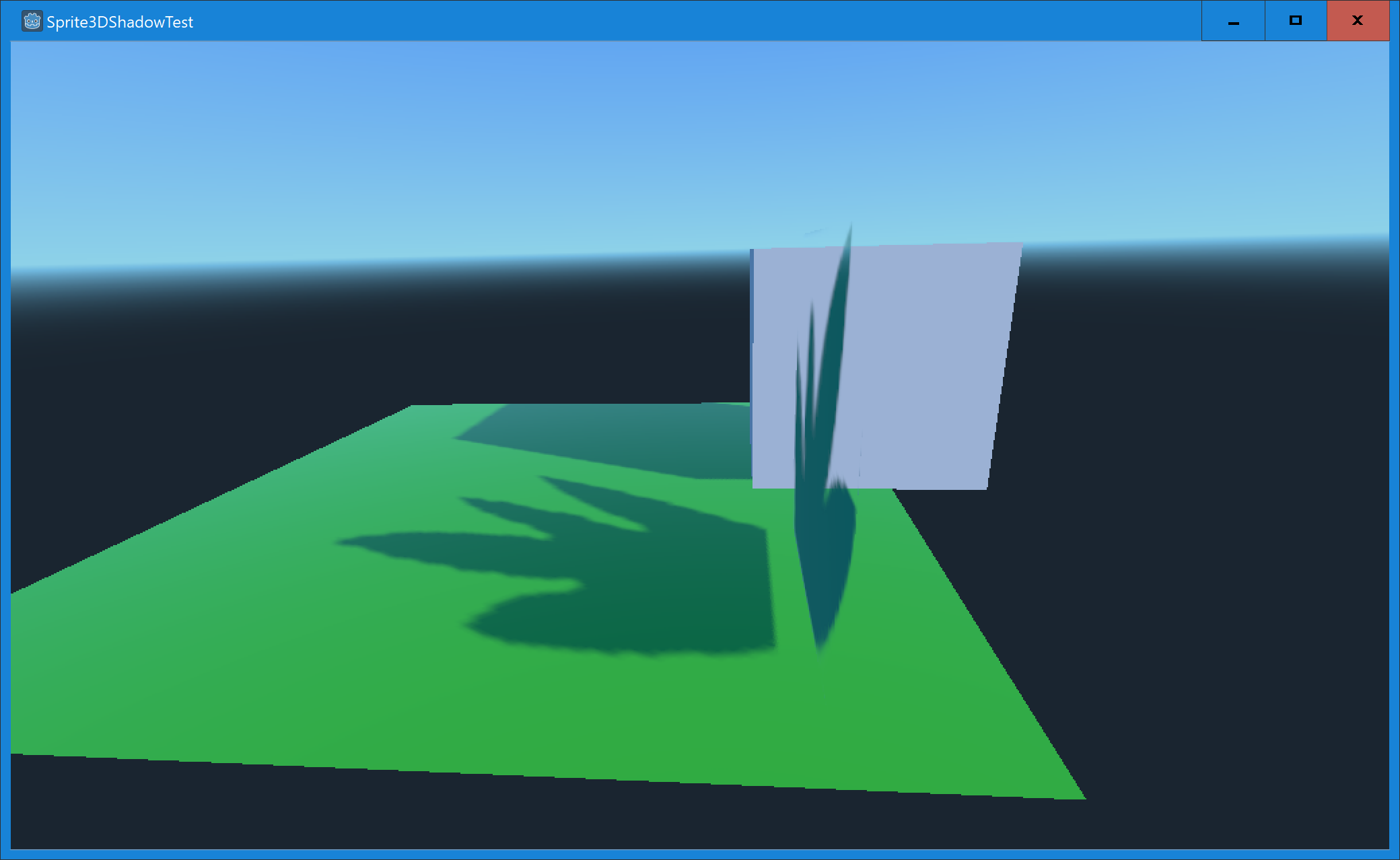
| bug,topic:rendering,topic:3d | low | Critical |
306,005,135 | TypeScript | In JS, aliasing of C.prototype should cause property assignments to add to the instance | ```ts
// from acorn
function Parser() {
/** @type {number} */
this.x = 1
}
var pp = Parser.prototype
pp.m = function () {
this.x = "should be an error"
return this.x
}
new Parser().m
```
**Expected behavior:**
`pp.m = function() ...` should add a method to the `Parser` instance. This should result in:
1. Error: can't assign string to property x of type number
2. No error on `new Parser().m`
**Actual behavior:**
No error on `this.x = "should be an error"` and an error on `new Parser().m` | Suggestion,Needs Proposal,checkJs,Domain: JavaScript | low | Critical |
306,028,878 | vue | Extending object syntax of v-on to support modifiers | ### What problem does this feature solve?
The object syntax of the v-on directive is vital in achieving conditional binding of event listeners, as shown in the discussions at https://github.com/vuejs/vue/issues/7349.
<div v-on="{ mouseover: condition ? handler : null }">
However, the current object syntax does not allow modifiers.
This feature request suggest that we extend the object syntax in the following way to allow modifiers.
<div v-on="{ click: { left: { prevent: condition ? leftClickHandler : null } }, mouseover: { stop: mouseoverHandler } }">
The above example would conditionally install leftClickHandler on "click.left.prevent" and mouseoverHandler on "mouseover.stop".
The embedded object notation is also conceptually consistent with the dot-notation already adopted in both function and inline syntax.
### What does the proposed API look like?
The proposed v-on object syntax would like like this, which is an extension of the current syntax.
<div v-on="{ click: { left: { prevent: condition ? leftClickHandler : null } }, mouseover: { stop: mouseoverHandler } }">
<!-- generated by vue-issues. DO NOT REMOVE --> | feature request | medium | Critical |
306,029,833 | flutter | flutter doctor - The resource loader cache does not have a loaded MUI entry . (HRESULT: 0x80073B01 Exception) | ## Steps to Reproduce
Running `flutter doctor` in PowerShell
## Logs
```
C:\Users\Pedro>flutter doctor
Checking Dart SDK version...
Downloading Dart SDK from Flutter engine ead227f118077d1f2b57842a32abaf105b573b8a...
Start-BitsTransfer : flutter doctor - 69/5000 The resource loader cache does not have a loaded MUI entry . (HRESULT: 0x80073B01 Exception)
En C:\Users\Pedro\flutter\bin\internal\update_dart_sdk.ps1: 47 Carácter: 1
+ Start-BitsTransfer -Source $dartSdkUrl -Destination $dartSdkZip
+ ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
+ CategoryInfo : NotSpecified: (:) [Start-BitsTransfer], COMException
+ FullyQualifiedErrorId : System.Runtime.InteropServices.COMException,Microsoft.BackgroundIntelligentTransfer.Mana
gement.NewBitsTransferCommand
Error: Unable to update Dart SDK. Retrying...
Esperando 2 segundos, presione CTRL+C para salir ...
```
## Flutter Doctor
--I can't see version due to this error | c: crash,tool,platform-windows,t: flutter doctor,a: first hour,P2,team-tool,triaged-tool | low | Critical |
306,035,798 | scrcpy | Thank you for this project :) | I have waited so long for an opensource alternative to some paid options. This is a big help to the community. Big thanks :) | wontfix | medium | Major |
306,052,900 | opencv | FindPNG overwrites settings of BuildZLIB | <!--
If you have a question rather than reporting a bug please go to http://answers.opencv.org where you get much faster responses.
If you need further assistance please read [How To Contribute](https://github.com/opencv/opencv/wiki/How_to_contribute).
This is a template helping you to create an issue which can be processed as quickly as possible. This is the bug reporting section for the OpenCV library.
-->
##### System information (version)
<!-- Example
- OpenCV => 3.1
- Operating System / Platform => Windows 64 Bit
- Compiler => Visual Studio 2015
-->
- OpenCV => 3.4.1
- Operating System / Platform => Ubuntu 16.04
- Compiler => GCC 5.4.0
##### Detailed description
When I configure cmake with the options: ``-DBUILD_ZLIB=ON -DWITH_PNG=ON -DBUILD_PNG=OFF``, the settings of `ZLIB` that are set here:
https://github.com/opencv/opencv/blob/master/cmake/OpenCVFindLibsGrfmt.cmake#L22
are overwritten by the call of ``FindPNG`` included performed here:
https://github.com/opencv/opencv/blob/master/cmake/OpenCVFindLibsGrfmt.cmake#L162
The reason is that `FindPNG`:
https://github.com/LuaDist/libgd/blob/master/cmake/modules/FindPNG.cmake#L18
includes `FindZLIB`:
https://github.com/Kitware/CMake/blob/master/Modules/FindZLIB.cmake
which does not check if ZLIB has already been found and simply overwrites corresponding variables, including the ``ZLIB_INCLUDE_DIRS``
Hence, when I finally build my project, the include directories for `zlib` are incorrect.
The current work-around is to use ``-DBUILD_ZLIB=ON -DWITH_PNG=ON -DBUILD_PNG=ON`` so that the png library is built, too. | priority: low,category: build/install | low | Critical |
306,058,616 | create-react-app | verifyPackageTree() errors when wrong version dependency is installed in any parent directory | ### Is this a bug report?
Yes
### Did you try recovering your dependencies?
No. This issue presents an argument that there may be a bug in the [verifyPackageTree.js](https://github.com/facebook/create-react-app/blob/next/packages/react-scripts/scripts/utils/verifyPackageTree.js) logic added in the `next` branch.
### Which terms did you search for in User Guide?
`version`, `dependenc`. This does not appear to be documented (and since it is internal, maybe it shouldn't be). There is discussion regarding documenting some of the packages related to this in issue in issue #4137 (suggested by @Timer [here](https://github.com/facebook/create-react-app/issues/4137#issuecomment-372003393)).
### Environment
```bash
$ node -v
v8.9.4
$ npm -v
5.7.1
$ yarn --version
1.5.1
```
Running on macOS `10.13.2`.
### Steps to Reproduce
I created a reproduction project here: https://github.com/newoga/create-react-app-issue-4167
The project is a simple node package that depends on a version of `jest` that is incompatible with `[email protected]`. The project also contains a directory (`cra-app`) that was generated by `create-react-app`. The `react-scripts` dependency in that sub-project has been updated to `2.0.0-next.47d2d941`.
1. `git clone https://github.com/newoga/create-react-app-issue-4167`
2. `cd create-react-app-issue-4167`
3. `yarn`
4. `yarn run`
### Expected Behavior
From a user perspective, the `yarn run` command should not error and the application should start. The user did not manually install an incompatible version of `jest` in the `create-react-app` generated project. The version of `jest` in the generated project's `node_modules` is the correct version and parent directories should not have an impact.
From a technical perspective, the `verifyPackageTree.js` logic should see that the `cra-app` project contains the correctly installed version of `jest` and stop checking parent directories. Parent directories should only be traversed if `jest` is not installed.
### Actual Behavior
An error occurs because parent directory depends on a version of `jest` that is incompatible with the version of `jest` that `create-react-app` generated project depends on.
### Reproducible Demo
https://github.com/newoga/create-react-app-issue-4167
**Edit:** Updated the issue number on the links. | issue: needs investigation | medium | Critical |
306,103,421 | TypeScript | Proposal: Easier Migration with Loose Mode in TypeScript Files | This issue is the dual of #23906.
# Background
TypeScript has always aimed at making migration from JavaScript as easy as possible; however, even today there exists a bit of an awkward gap where you must rewrite your JavaScript code to put it in a state where it's "ready" to be converted to TypeScript. As an example, users might start annotating their code with JSDoc to get better analysis in `.js` files, but once they switch their file extensions to `.ts`, TypeScript pretends like it doesn't understand those annotations anymore.
# Proposal
TypeScript can provide a new "loose" mode (which we'll refer to as `looseTs`). This loose mode can be seen as a hybrid between the `allowJs`/Salsa language service support for JavaScript, along with TypeScript itself.
Let's explore what would this experience would look like.
## Special JavaScript Declarations Are Allowed
TypeScript would understand certain constructs that are currently special cased in JS files. For example, the following construct would create what TypeScript effectively sees as a constructor.
```ts
function Foo() {
this.x = 10;
this.y = 20;
this.z = 30;
}
new Foo().x + new Foo().y + new Foo().z // all well-typed as 'number'
```
Our language service could provide tooling to help refactor this to a more canonical sort of class.
## Type Annotations & Declarations
All TypeScript constructs would continue to work, but so would JSDoc annotations & declarations.
```ts
/**
* @typedef {PersonName} string
*/
export interface Person {
// valid to reference JSDoc declarations in the same file
name: PersonName;
}
```
TypeScript constructs would always take precedent over their JSDoc counterparts when they potentially conflict. For example, the following provides an error at worst, but will always consider `x` to be a `string`:
```ts
/**
* @type {number}
*/
var x: string;
```
## Class Properties
Today, TypeScript requires all classes to have property declarations.
Future versions of ECMAScript might *allow* property declarations.
The story here is: pick one. If classes contain no property declarations, then we'll fall back to Salsa-style inference from the constructor body. If any property declarations are present, then any access to `this` with a member that hasn't been declared is an error.
```ts
// Good!
class AllDeclared {
a: number;
constructor() {
this.a = 0;
}
}
// Good!
// Also, users get a quick fix.
class OnlyInitialized {
constructor() {
this.a = 0;
}
}
// Bad!
class MixedDeclarationsAndInitialization {
a: number;
constructor() {
this.a = 10;
this.b = "hallo";
}
}
```
We can provide tooling to help migrate users to TypeScript code that declares all of the initialized properties.
## Best-Effort Completions
Like in Salsa, we should provide loose completions for things like `any` by default. This might be editor-configurable, but see #5334 for some more discussion.
## More?
Your ideas here!
# Drawbacks
One argument is that this could potentially dilute the value of the language, similar to concerns around `// @ts-ignore`.
Another related argument has to do with cognitive overhead. Our heuristics around understanding JavaScript constructs in Salsa (our JS language service) feels somewhat opaque. While we can document what special constructs we support, TypeScript's current model is significantly simpler. It might not be clear what features "proper TypeScript" actually supports (i.e. what exactly are you giving up when you turn off `looseTs`?)
# Alternatives
We may want to consider alternatives before we commit to something like this.
## Type Annotations in JavaScript Files
This proposal has proposed the idea of understanding Salsa/JS constructs in `.ts` files, but one could approach it from the other direction: Salsa could support type annotations in `.js` files instead under a specific mode. Our main concern here has been the conflation between what is possible in JavaScript today, and what constructs are specific to TypeScript. Additionally, providing non-standard constructs in `.js` files might give users the wrong impression over what we're trying to achieve (to clarify, in this issue, we're here to provide tooling for JavaScript users and make it easier to migrate to TypeScript).
## Tooling Tooling Tooling Tooling
Now that we have suggestion diagnostics, we can potentially provide suggestions & tooling to just fix "legacy" JS constructs in the language service once you've moved to TypeScript. The counter-point here is that users will still likely experience the "sea of red" problem when you first switch a file over to `.ts`. | Suggestion,Awaiting More Feedback | low | Critical |
306,113,248 | godot | Shaders rendered on a transparent viewport are darker | **Godot version:**
Godot Engine v3.0.2.stable.official
**OS/device including version:**
Windows 10 Home v1709 (Fall Creator's Update); Nvidia GTX 660 (I don't believe this is specific to my GPU, though)
**Issue description:**
Shaders that get rendered on a transparent viewport (with nothing behind them) end up darker than they normally should. This can be solved by moving the object with a shader outside of any custom viewport, but I imagine that would end up getting messy quick, if viewports are being relied upon in some way.
What's expected:
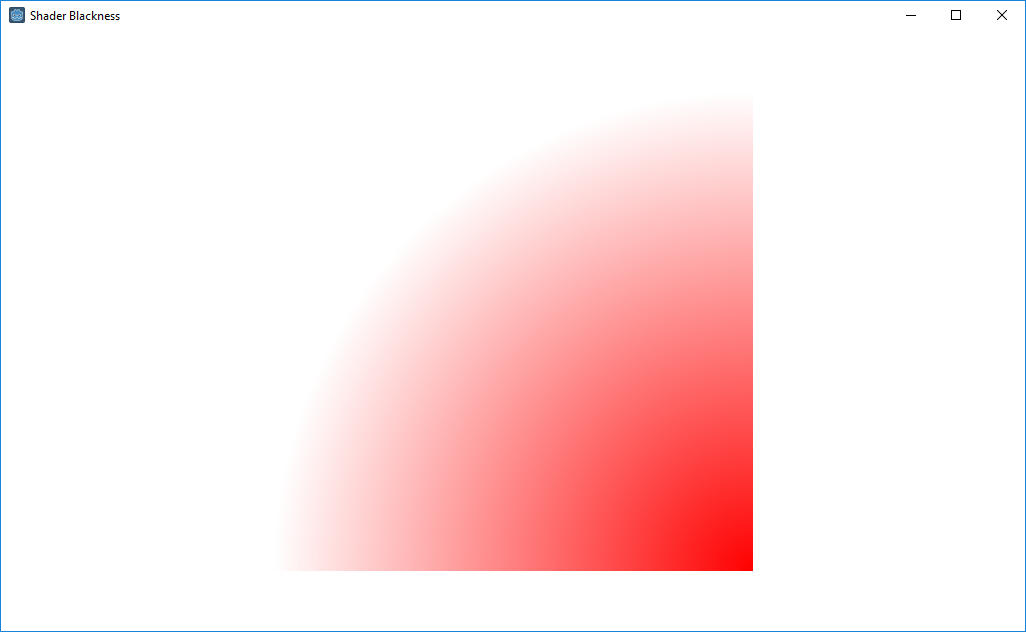
What actually happened:
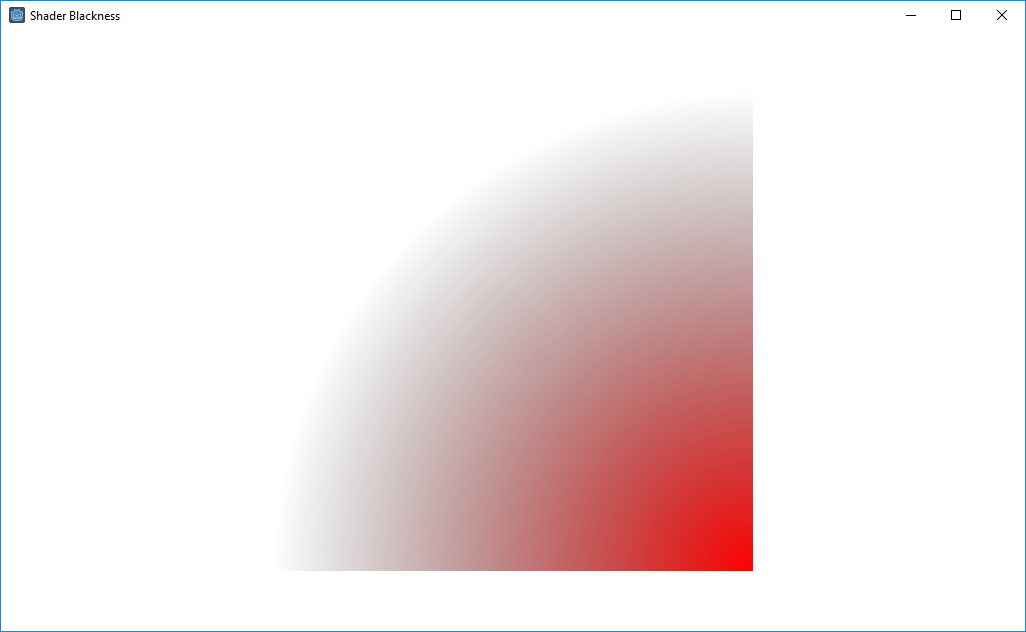
I believe this has to do with how the transparent viewport itself is handled, as having something behind the shader under the viewport will cause the shader to not be dark. (Try moving the ColorRect to under the Viewport, above ShaderPanel, in the reproduction project to see what I mean.)
**Steps to reproduce:**
1. Create a viewport, and enable the "Transparent BG" option, as well as setting up the size.
2. Create a Panel node (or really any node that supports a shader, I just use a Panel node) under that viewport.
3. Give the Panel node a shader that will, in some way, return some transparent pixels. The one included in the reproduction project below (shader.tres) will work for this purpose, and should show the effect fairly well due to creating a red-to-transparent gradient like in the images above.
4. Create a sprite, and set its texture to the ViewportTexture of the viewport.
5. (Optional, but makes the effect easier to see) Make a ColorRect, and place it behind the Sprite.
6. When the panel is under the Viewport, the image that the shader makes should be darker than expected. Moving the panel out of the Viewport's children will show the expected result.
**Minimal reproduction project:**
[Shader Blackness.zip](https://github.com/godotengine/godot/files/1821096/Shader.Blackness.zip)
| topic:rendering,confirmed,documentation,topic:shaders | medium | Critical |
306,127,825 | TypeScript | Proposal: Overload Function.bind for when no argArray is provided. | # Background
Currently the `Function.bind` type definition defines only one signature:
```typescript
bind(this: Function, thisArg: any, ...argArray: any[]): any;
```
This destroys typing information on the bound function. Given difficulties in generating a new type for the bound function (the number of arguments has changed) this makes sense. However, it's very common to bind functions without using the `argArray` argument to `bind`. In this case a better solution can be made.
# Proposal
I propose that `bind` be overloaded with a second signature:
```typescript
bind<T extends Function>(this: T, thisArg: any): T;
bind(this: Function, thisArg: any, ...argArray: any[]): any;
```
# Example
To use a real world example on how this improves TypeScript I'll pull something from a personal project.
Types used:
```typescript
type SocketMsg = Guess | StateMsg;
type ChannelCallback<T> = (payload: T, ref: Ref, joinRef: Ref) => any;
export class Channel<T> {
// ...
on(event: ChannelEvent, callback: ChannelCallback<T>): void;
// ...
}
```
```typescript
export default class GuessView extends Component<GuessProps, GuessState> {
channel: Channel<SocketMsg>;
connectSocket() {
...
this.channel.on("guess", this.gameGuess.bind(this));
}
gameGuess(guess: Guess) {
let newState = Object.assign({}, this.state);
newState.guesses.push(guess);
this.setState(newState);
}
}
```
Under the current `bind` signature a change to `gameGuess` such that it accepts a different type would produce no error:
```typescript
connectSocket() {
...
this.channel.on("guess", this.gameGuess.bind(this)); // Now being provided the wrong type of function
}
gameGuess(guess: number) {
// ...
```
But with `bind` utilizing a special definition for the case of only using the `thisArg` argument - the above example would produce an error.
> Argument of type '(guess: number) => void' is not assignable to parameter of type 'ChannelCallback<SocketMsg>'. Types of parameters 'guess' and 'payload' are incompatible. Type 'SocketMsg' is not assignable to type 'number'. Type 'Guess' is not assignable to type 'number'. | Suggestion,In Discussion | low | Critical |
306,146,135 | neovim | win: jobwait(): use WaitForSingleObject | - `jobwait()` (which depends on `process_wait()` internally) needs to be revisited on Windows.
Maybe it should be implemented using `WaitForSingleObject`. https://stackoverflow.com/a/2153846/
- ~~But `process_stop()` was changed in https://github.com/neovim/neovim/pull/8107 , so maybe `jobwait()` works better after that.~~
- Related: the "proper"/non-racey (Linux) way to kill a process tree is to [use cgroups](https://news.ycombinator.com/item?id=20599575).
Is this rare test failure related?
```
[ ERROR ] C:/projects/neovim/test/functional\api\proc_spec.lua @ 17: api nvim_get_proc_children returns child process ids
test\functional\helpers.lua:323:
retry() attempts: 434
C:/projects/neovim/test/functional\api\proc_spec.lua:22: Expected objects to be the same.
Passed in:
(number) 3
Expected:
(number) 1
stack traceback:
``` | platform:windows,job-control,needs:discussion,complexity:low,system | low | Critical |
306,159,335 | rust | Incorrect warning: Unnecessary unsafe block | 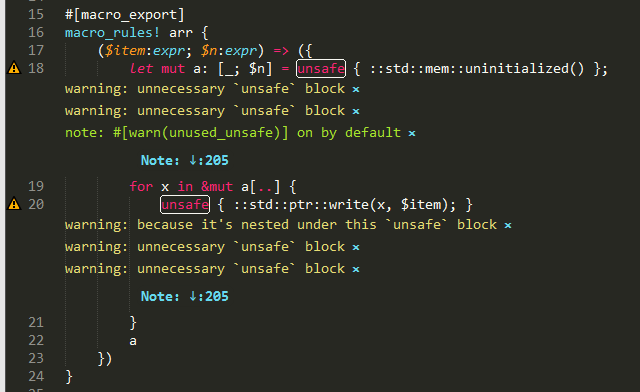
This warning is incorrect. It says for both unsafe blocks that they are unnecessary, and when either of the unsafe keywords is removed, it gives an error (of course).
It wrongly says that the first block is nested under the second unsafe block.
Before (nightly at end of January) this warning didn't occur.
| C-enhancement,A-diagnostics,T-compiler | low | Critical |
306,165,229 | opencv | ProjectPoints incorrect result w/Java bindings and z-coordinate small |
##### System information (version)
- OpenCV => java_340
- Operating System / Platform => win64
- Compiler => javac 1.8
##### Detailed description
When I run projectPoints function using java code below with most simple inputs I get what I believe is incorrect answer when z is small. Here is result that shows problem. I find this works in python correctly.
(x,y,z)= [{0.0, 1.0, 1.0}, {0.0, 1.0, 5.0}, {0.0, 1.0, 10.0}]
(u,v)= [{0.0, 78.5398178100586}, {0.0, 19.73955535888672}, {0.0, 9.966865539550781}]
should be
(u,v)=[{0.0, 100.0}, {0.0, 20.0}, {0.0, 10.0}]
##### Steps to reproduce
```
public static void main(String[] args) {
setDllLibraryPath("C:/aaa_eric/code/lib/x64");
System.loadLibrary(Core.NATIVE_LIBRARY_NAME); //340
MatOfPoint3f objectPts3f = new MatOfPoint3f(
new Point3(0.0,1.0, 1.0),
new Point3(0.0,1.0, 5.0),
new Point3(0.0,1.0,10.0));
MatOfPoint2f imagePts2f = new MatOfPoint2f();
Mat rVec = Mat.zeros(3,1, CvType.CV_64F);
Mat tVec = Mat.zeros(3,1, CvType.CV_64F);
//camera matrix, no distortion
Mat kMat = Mat.zeros(3, 3, CvType.CV_64F);
kMat.put(0, 0,
100.0, 0.0, 0.0,
0.0, 100.0, 0.0,
0.0, 0.0, 1.0);
Mat dMat = Mat.zeros(4, 1, CvType.CV_64F);
Calib3d.projectPoints(objectPts3f, imagePts2f, rVec, tVec, kMat, dMat);
System.out.println(objectPts3f.toList());
System.out.println(imagePts2f.toList());
}
``` | category: calib3d,category: java bindings | low | Minor |
306,184,989 | pytorch | Change THCudaCheck to suggest that device-side asserts likely mean that you have out of bound indices | Hi
I have written the following code to train my model, after a few iterations it stops throwing a similar error as shown in the image. Note that error is similar but not same, it changes everytime. I have read all the answers here and pytorch forum for the similar errors, none of them works. Please help ...
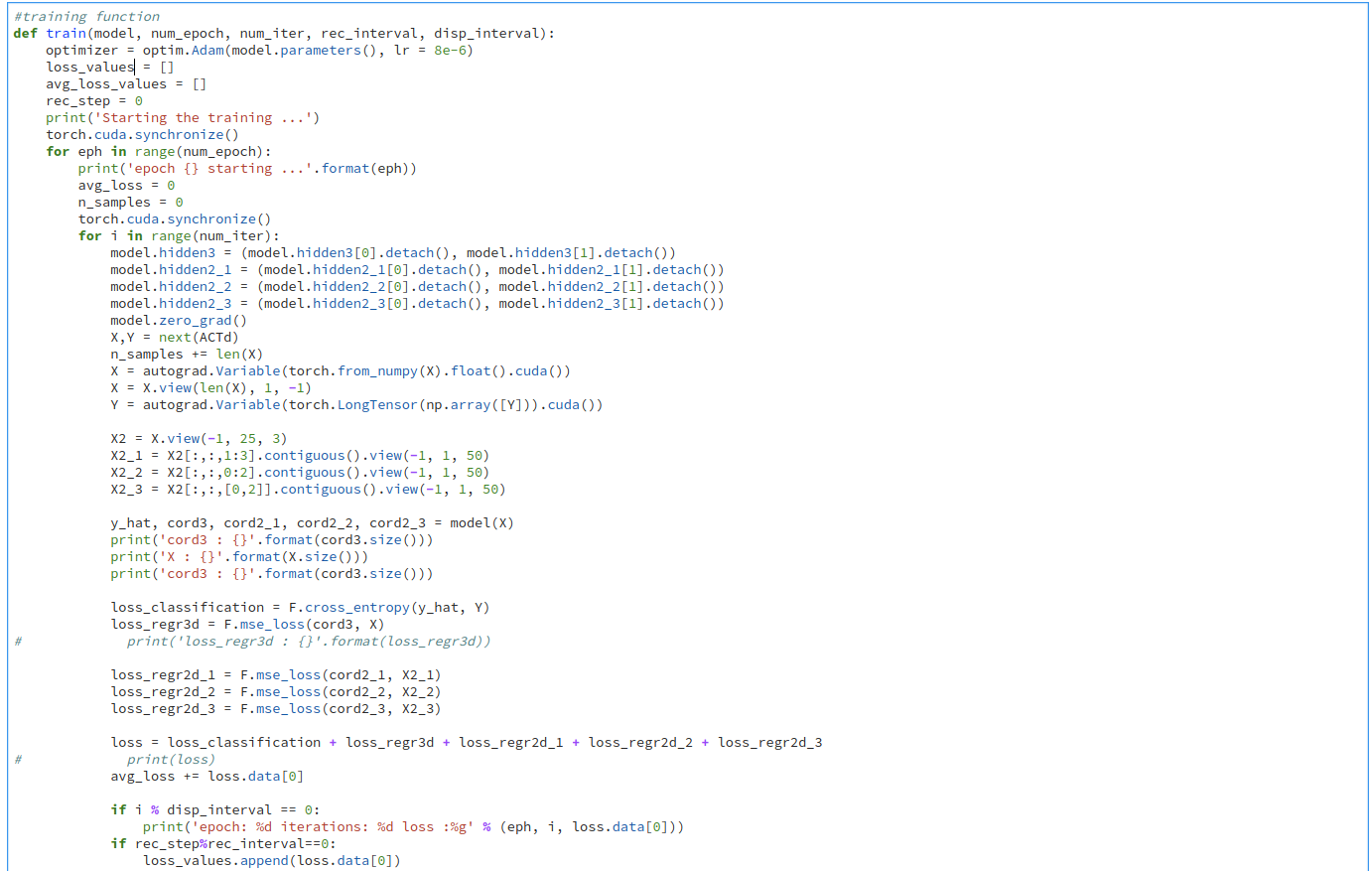
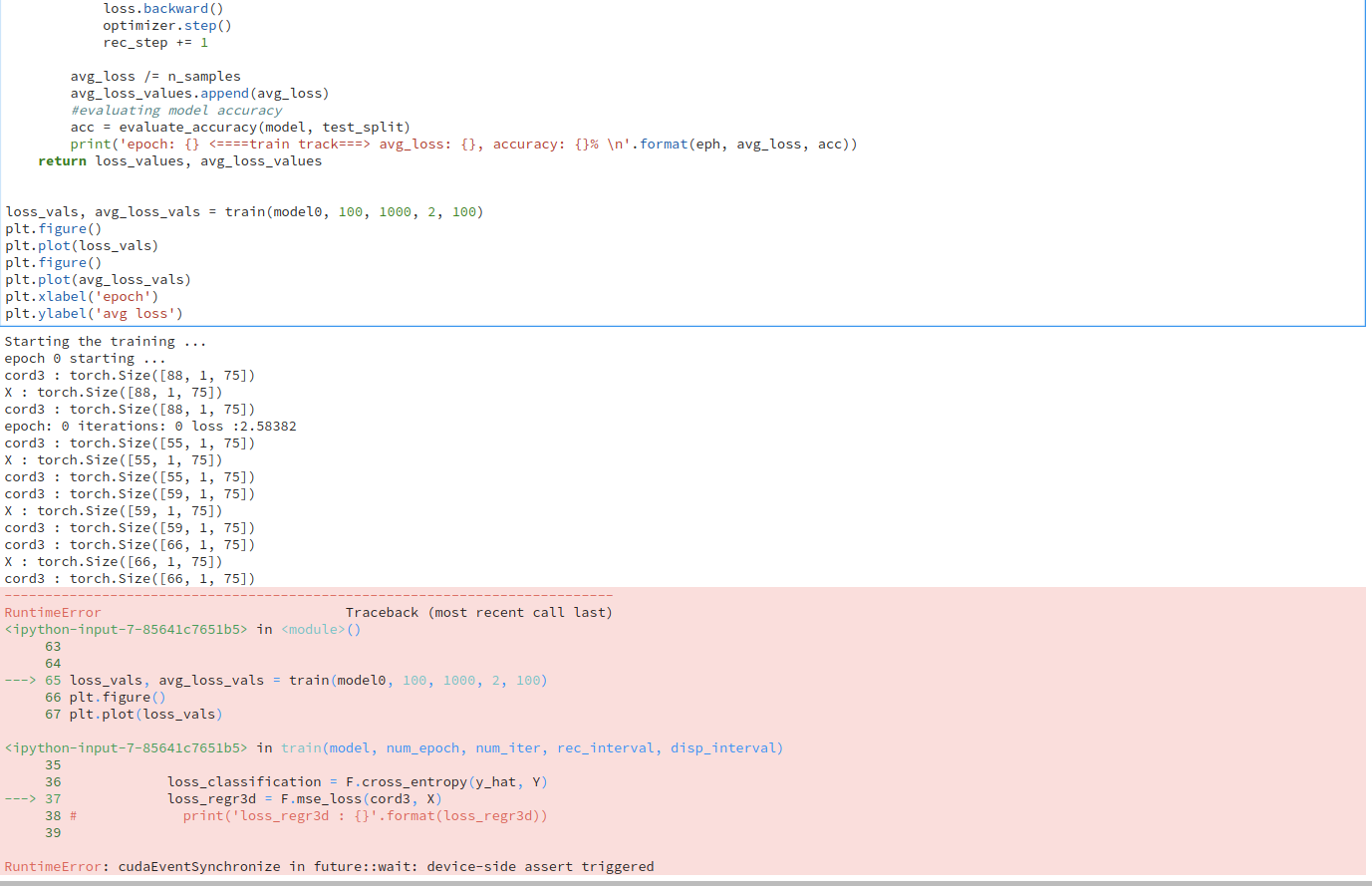
**Note that it is running for few iterations hence the starting few outputs**
Thank you. | module: cuda,module: error checking,triaged,module: assert failure,small | low | Critical |
306,192,987 | TypeScript | Removing "private" modifier from types | [Feature suggestion]
How about this (for Typescript 2.8+):
```ts
type Friend<T> = {
-private [P in keyof T]: T[P]
}
``` | Suggestion,Awaiting More Feedback | high | Critical |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.