id
int64 393k
2.82B
| repo
stringclasses 68
values | title
stringlengths 1
936
| body
stringlengths 0
256k
⌀ | labels
stringlengths 2
508
| priority
stringclasses 3
values | severity
stringclasses 3
values |
---|---|---|---|---|---|---|
240,041,524 |
TypeScript
|
Narrow type of variable when declared as literal (also tuples)
|
<!-- SUGGESTIONS: See https://github.com/Microsoft/TypeScript-wiki/blob/master/Writing-Good-Design-Proposals.md -->
**TypeScript Version:** 2.4.1
**Code**
The following doesn't compile, because `x` has inferred type `string`. I think it would be helpful if it did, but I still want `x` to have inferred type `string`:
```ts
function blah(arg: "foo" | "bar") {
}
let x = "foo";
blah(x);
x = "something else";
```
**Desired behavior:**
This would compile.
**Actual behavior:**
`demo.ts(4,6): error TS2345: Argument of type 'string' is not assignable to parameter of type '"foo" | "bar"'.`
**Suggestion**
The type of `x` should be inferred to be `string`, but it should be narrowed to `"foo"` via flow-sensitive typing, much like the below program:
```ts
function blah(arg: "foo" | "bar") {
}
let x = "foo";
if (x != "foo") throw "impossible"; // NOTE: codegen would NOT insert this
blah(x);
x = "something else";
```
Note that *this* program currently works as desired: `x` is still inferred to have type `string`, but the guard immediately narrows it to type `"foo"`. The assignment after the function call widens `x`'s type back to `string`.
My suggestion is for TS to treat declarations that assign variables to literals (as in the first example) to automatically perform this flow-sensitive type narrowing, without the need for an unnecessary guard.
I'm suggesting it only for declarations, not general assignments (so only with `let`, `var`, and `const`) or other operations. In addition, I'm only suggesting it for assignments to literal values (literal strings or literal numbers) without function calls, operations, or casts.
**Syntax Changes**
Nothing changes in syntax.
**Code Generation**
Nothing changes in code generation.
**Semantic Changes**
Additional flow-sensitive type narrowing occurs when variables are declared as literals, essentially making the existing
```ts
let x = "foo";
```
behave the same as (in checking, but not in codegen)
```ts
let x = "foo";
if (x != "foo") throw "unreachable";
```
**Reverse Compatibility**
Due to `x` being assigned a more-precise type than it was in the past, some code is now marked as unreachable/invalid that previously passed:
```ts
let x = "foo";
if (x == "bar") { // error TS2365: Operator '==' cannot be applied to types '"foo"' and '"blah"'.
// ...
}
```
The error is correct (in that the comparison could never succeed) but it's still possibly undesirable.
I don't know how commonly this occurs in production code for it to be a problem. If it is common, this change could be put behind a flag, or there could be adjustments made to these error cases so that the narrowing doesn't occur (or still narrows, but won't cause complaints from the compiler in these error cases).
If the existing behavior is strongly needed, then the change can be circumvented by using an `as` cast or an indirection through a function, although these are a little awkward:
```ts
let x = "foo" as string;
let x = (() => "foo")()
```
## Tuples and Object Literals
It would be helpful if this extended also to literal objects or literal arrays (as tuples).
For example, the following could compile:
```ts
function blah(arg: [number, number]) {
}
let x = [1, 3];
blah(x);
x = [1, 2, 3];
```
with `x` again having inferred type `number[]` but being flow-typed to `[number, number]`. The same basic considerations apply here as above.
Similarly, it could be useful for objects to have similar flow-typing, although I'm not sure if this introduces new soundness holes:
```ts
function get(): number {
return 0;
}
function blah(arg: {a: "foo" | "bar", b: number}) {
// (...)
}
let y = get(); // y: number
let x = {a: "foo", b: y}; // x: {a: string, b: number}
// x is narrowed to {a: "foo", b: number}
blah(x); // this compiles due to x's narrowed type
x.a = "something else"; // this is accepted, because x: {a: string, b: number}.
```
See https://github.com/Microsoft/TypeScript/issues/16276 and https://github.com/Microsoft/TypeScript/issues/16360 and probably others for related but different approaches to take here.
|
Suggestion,Needs Proposal
|
medium
|
Critical
|
240,059,045 |
youtube-dl
|
[dnvod] Site support request
|
## Please follow the guide below
- You will be asked some questions and requested to provide some information, please read them **carefully** and answer honestly
- Put an `x` into all the boxes [ ] relevant to your *issue* (like that [x])
- Use *Preview* tab to see how your issue will actually look like
---
### Make sure you are using the *latest* version: run `youtube-dl --version` and ensure your version is *2017.07.02*. If it's not read [this FAQ entry](https://github.com/rg3/youtube-dl/blob/master/README.md#how-do-i-update-youtube-dl) and update. Issues with outdated version will be rejected.
- [x] I've **verified** and **I assure** that I'm running youtube-dl **2017.07.02**
### Before submitting an *issue* make sure you have:
- [x] At least skimmed through [README](https://github.com/rg3/youtube-dl/blob/master/README.md) and **most notably** [FAQ](https://github.com/rg3/youtube-dl#faq) and [BUGS](https://github.com/rg3/youtube-dl#bugs) sections
- [x] [Searched](https://github.com/rg3/youtube-dl/search?type=Issues) the bugtracker for similar issues including closed ones
### What is the purpose of your *issue*?
- [ ] Bug report (encountered problems with youtube-dl)
- [x] Site support request (request for adding support for a new site)
- [ ] Feature request (request for a new functionality)
- [ ] Question
- [ ] Other
---
### If the purpose of this *issue* is a *site support request* please provide all kinds of example URLs support for which should be included (replace following example URLs by **yours**):
- Single video: http://www.dnvod.tv/Movie/Readyplay.aspx?id=wnLClIqsPII%3d
Note that **youtube-dl does not support sites dedicated to [copyright infringement](https://github.com/rg3/youtube-dl#can-you-add-support-for-this-anime-video-site-or-site-which-shows-current-movies-for-free)**. In order for site support request to be accepted all provided example URLs should not violate any copyrights.
---
|
site-support-request
|
low
|
Critical
|
240,120,384 |
youtube-dl
|
[Go] Unnecessarily verbose FFmpeg output
|
## Please follow the guide below
- You will be asked some questions and requested to provide some information, please read them **carefully** and answer honestly
- Put an `x` into all the boxes [ ] relevant to your *issue* (like that [x])
- Use *Preview* tab to see how your issue will actually look like
---
### Make sure you are using the *latest* version: run `youtube-dl --version` and ensure your version is *2017.07.02*. If it's not read [this FAQ entry](https://github.com/rg3/youtube-dl/blob/master/README.md#how-do-i-update-youtube-dl) and update. Issues with outdated version will be rejected.
- [x] I've **verified** and **I assure** that I'm running youtube-dl **2017.07.02**
### Before submitting an *issue* make sure you have:
- [x] At least skimmed through [README](https://github.com/rg3/youtube-dl/blob/master/README.md) and **most notably** [FAQ](https://github.com/rg3/youtube-dl#faq) and [BUGS](https://github.com/rg3/youtube-dl#bugs) sections
- [x] [Searched](https://github.com/rg3/youtube-dl/search?type=Issues) the bugtracker for similar issues including closed ones
### What is the purpose of your *issue*?
- [x] Bug report (encountered problems with youtube-dl)
- [ ] Site support request (request for adding support for a new site)
- [ ] Feature request (request for a new functionality)
- [ ] Question
- [ ] Other
---
### The following sections concretize particular purposed issues, you can erase any section (the contents between triple ---) not applicable to your *issue*
---
### If the purpose of this *issue* is a *bug report*, *site support request* or you are not completely sure provide the full verbose output as follows:
Add `-v` flag to **your command line** you run youtube-dl with, copy the **whole** output and insert it here. It should look similar to one below (replace it with **your** log inserted between triple ```):
Since adding `-v` generates additional console output, I will provide both verbose and non-verbose console output:
<details>
<summary>Verbose log</summary>
```
nyuszika7h@panlyn ~ % youtube-dl -v --ignore-config 'http://watchdisneychannel.go.com/andi-mack/video/vdka3727119/01/01-13'
[debug] System config: []
[debug] User config: []
[debug] Custom config: []
[debug] Command-line args: ['-v', '--ignore-config', 'http://watchdisneychannel.go.com/andi-mack/video/vdka3727119/01/01-13']
[debug] Encodings: locale UTF-8, fs utf-8, out UTF-8, pref UTF-8
[debug] youtube-dl version 2017.07.02
[debug] Python version 3.6.1+ - Linux-4.4.0-43-Microsoft-x86_64-with-Ubuntu-16.04-xenial
[debug] exe versions: ffmpeg 3.3.2-1, ffprobe 3.3.2-1, rtmpdump 2.4
[debug] Proxy map: {}
[Go] vdka3727119: Downloading JSON metadata
[debug] Using fake IP 3.210.223.193 (US) as X-Forwarded-For.
[Go] VDKA3727119: Downloading JSON metadata
[Go] VDKA3727119: Downloading m3u8 information
[debug] Invoking downloader on 'http://content-aeuf1.uplynk.com/b4594ce3d0b7447a9e43dbfa2128f450/j.m3u8?exp=1499075032&ct=a&oid=21885d134fa441488df8e1d7acee8c66&eid=10087805&iph=5f72106d5f10622d58c00a02db5f9015572ad88a12d556f4ede6918b51c26703&rays=jihgfedcb&euid=C5B4DF9A-2D28-42FE-9841-4078C1C2A82C_000_0_001_lf_03-06-00_NA&cdn=ec&stgcfg=datg&pp2ip=0&ddp=1&sig=c288780569bf6898bf9ad1d8d8246a929571bfde637f92ffdf44cacd49d2673d&pbs=1f50607d8d064a4eb22e72db53bff2f5'
[download] Destination: S1 E1 - 13-VDKA3727119.mp4
[debug] ffmpeg command line: ffmpeg -y -loglevel verbose -headers 'User-Agent: Mozilla/5.0 (X11; Linux x86_64; rv:10.0) Gecko/20150101 Firefox/47.0 (Chrome)
Accept-Charset: ISO-8859-1,utf-8;q=0.7,*;q=0.7
Accept: text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8
Accept-Encoding: gzip, deflate
Accept-Language: en-us,en;q=0.5
X-Forwarded-For: 3.210.223.193
' -i 'http://content-aeuf1.uplynk.com/b4594ce3d0b7447a9e43dbfa2128f450/j.m3u8?exp=1499075032&ct=a&oid=21885d134fa441488df8e1d7acee8c66&eid=10087805&iph=5f72106d5f10622d58c00a02db5f9015572ad88a12d556f4ede6918b51c26703&rays=jihgfedcb&euid=C5B4DF9A-2D28-42FE-9841-4078C1C2A82C_000_0_001_lf_03-06-00_NA&cdn=ec&stgcfg=datg&pp2ip=0&ddp=1&sig=c288780569bf6898bf9ad1d8d8246a929571bfde637f92ffdf44cacd49d2673d&pbs=1f50607d8d064a4eb22e72db53bff2f5' -c copy -f mp4 'file:S1 E1 - 13-VDKA3727119.mp4.part'
ffmpeg version 3.3.2-1~16.04.york1 Copyright (c) 2000-2017 the FFmpeg developers
built with gcc 5.4.0 (Ubuntu 5.4.0-6ubuntu1~16.04.4) 20160609
configuration: --prefix=/usr --extra-version='1~16.04.york1' --toolchain=hardened --libdir=/usr/lib/x86_64-linux-gnu --incdir=/usr/include/x86_64-linux-gnu --enable-gpl --disable-stripping --enable-avresample --enable-avisynth --enable-gnutls --enable-ladspa --enable-libass --enable-libbluray --enable-libbs2b --enable-libcaca --enable-libcdio --enable-libflite --enable-libfontconfig --enable-libfreetype --enable-libfribidi --enable-libgme --enable-libgsm --enable-libmp3lame --enable-libopenjpeg --enable-libopenmpt --enable-libopus --enable-libpulse --enable-librubberband --enable-libshine --enable-libsnappy --enable-libsoxr --enable-libspeex --enable-libssh --enable-libtheora --enable-libtwolame --enable-libvorbis --enable-libvpx --enable-libwavpack --enable-libwebp --enable-libx265 --enable-libxvid --enable-libzmq --enable-libzvbi --enable-omx --enable-openal --enable-opengl --enable-sdl2 --enable-libdc1394 --enable-libiec61883 --enable-chromaprint --enable-frei0r --enable-libopencv --enable-libx264 --enable-shared
libavutil 55. 58.100 / 55. 58.100
libavcodec 57. 89.100 / 57. 89.100
libavformat 57. 71.100 / 57. 71.100
libavdevice 57. 6.100 / 57. 6.100
libavfilter 6. 82.100 / 6. 82.100
libavresample 3. 5. 0 / 3. 5. 0
libswscale 4. 6.100 / 4. 6.100
libswresample 2. 7.100 / 2. 7.100
libpostproc 54. 5.100 / 54. 5.100
[hls,applehttp @ 0x7ffff7f0d8a0] HLS request for url 'http://x-disney-datg-stgec.uplynk.com/80C078/ausc/slices/b45/21885d134fa441488df8e1d7acee8c66/b4594ce3d0b7447a9e43dbfa2128f450/J00000000.ts?pbs=1f50607d8d064a4eb22e72db53bff2f5&euid=C5B4DF9A-2D28-42FE-9841-4078C1C2A82C_000_0_001_lf_03-06-00_NA&si=0', offset 0, playlist 0
[hls,applehttp @ 0x7ffff7f0d8a0] Opening 'https://content-aeuf1.uplynk.com/check2?b=b4594ce3d0b7447a9e43dbfa2128f450&v=b4594ce3d0b7447a9e43dbfa2128f450&r=j&pbs=1f50607d8d064a4eb22e72db53bff2f5' for reading
[hls,applehttp @ 0x7ffff7f0d8a0] Opening 'crypto+http://x-disney-datg-stgec.uplynk.com/80C078/ausc/slices/b45/21885d134fa441488df8e1d7acee8c66/b4594ce3d0b7447a9e43dbfa2128f450/J00000000.ts?pbs=1f50607d8d064a4eb22e72db53bff2f5&euid=C5B4DF9A-2D28-42FE-9841-4078C1C2A82C_000_0_001_lf_03-06-00_NA&si=0' for reading
[mpegts @ 0x7ffff7f5d5a0] parser not found for codec timed_id3, packets or times may be invalid.
[h264 @ 0x7ffff7f5dce0] Reinit context to 1280x720, pix_fmt: yuv420p
Input #0, hls,applehttp, from 'http://content-aeuf1.uplynk.com/b4594ce3d0b7447a9e43dbfa2128f450/j.m3u8?exp=1499075032&ct=a&oid=21885d134fa441488df8e1d7acee8c66&eid=10087805&iph=5f72106d5f10622d58c00a02db5f9015572ad88a12d556f4ede6918b51c26703&rays=jihgfedcb&euid=C5B4DF9A-2D28-42FE-9841-4078C1C2A82C_000_0_001_lf_03-06-00_NA&cdn=ec&stgcfg=datg&pp2ip=0&ddp=1&sig=c288780569bf6898bf9ad1d8d8246a929571bfde637f92ffdf44cacd49d2673d&pbs=1f50607d8d064a4eb22e72db53bff2f5':
Duration: 00:24:59.63, start: 0.000000, bitrate: 0 kb/s
Program 0
Metadata:
variant_bitrate : 0
Stream #0:0: Audio: aac (HE-AAC) ([15][0][0][0] / 0x000F), 48000 Hz, stereo, fltp
Metadata:
variant_bitrate : 0
Stream #0:1: Video: h264 (High), 1 reference frame ([27][0][0][0] / 0x001B), yuv420p(left), 1280x720, 23.98 tbr, 90k tbn, 48 tbc
Metadata:
variant_bitrate : 0
Stream #0:2: Data: timed_id3 (ID3 / 0x20334449)
Metadata:
variant_bitrate : 0
Output #0, mp4, to 'file:S1 E1 - 13-VDKA3727119.mp4.part':
Metadata:
encoder : Lavf57.71.100
Stream #0:0: Video: h264 (High), 1 reference frame ([33][0][0][0] / 0x0021), yuv420p(left), 1280x720 (0x0), q=2-31, 23.98 tbr, 90k tbn, 90k tbc
Metadata:
variant_bitrate : 0
Stream #0:1: Audio: aac (HE-AAC) ([64][0][0][0] / 0x0040), 48000 Hz, stereo, fltp
Metadata:
variant_bitrate : 0
Stream mapping:
Stream #0:1 -> #0:0 (copy)
Stream #0:0 -> #0:1 (copy)
Press [q] to stop, [?] for help
Automatically inserted bitstream filter 'aac_adtstoasc'; args=''
[hls,applehttp @ 0x7ffff7f0d8a0] HLS request for url 'http://x-disney-datg-stgec.uplynk.com/80C078/ausc/slices/b45/21885d134fa441488df8e1d7acee8c66/b4594ce3d0b7447a9e43dbfa2128f450/J00000001.ts?pbs=1f50607d8d064a4eb22e72db53bff2f5&euid=C5B4DF9A-2D28-42FE-9841-4078C1C2A82C_000_0_001_lf_03-06-00_NA&si=0', offset 0, playlist 0
[hls,applehttp @ 0x7ffff7f0d8a0] Opening 'crypto+http://x-disney-datg-stgec.uplynk.com/80C078/ausc/slices/b45/21885d134fa441488df8e1d7acee8c66/b4594ce3d0b7447a9e43dbfa2128f450/J00000001.ts?pbs=1f50607d8d064a4eb22e72db53bff2f5&euid=C5B4DF9A-2D28-42FE-9841-4078C1C2A82C_000_0_001_lf_03-06-00_NA&si=0' for reading
[hls,applehttp @ 0x7ffff7f0d8a0] HLS request for url 'http://x-disney-datg-stgec.uplynk.com/80C078/ausc/slices/b45/21885d134fa441488df8e1d7acee8c66/b4594ce3d0b7447a9e43dbfa2128f450/J00000002.ts?pbs=1f50607d8d064a4eb22e72db53bff2f5&euid=C5B4DF9A-2D28-42FE-9841-4078C1C2A82C_000_0_001_lf_03-06-00_NA&si=0', offset 0, playlist 0
[hls,applehttp @ 0x7ffff7f0d8a0] Opening 'crypto+http://x-disney-datg-stgec.uplynk.com/80C078/ausc/slices/b45/21885d134fa441488df8e1d7acee8c66/b4594ce3d0b7447a9e43dbfa2128f450/J00000002.ts?pbs=1f50607d8d064a4eb22e72db53bff2f5&euid=C5B4DF9A-2D28-42FE-9841-4078C1C2A82C_000_0_001_lf_03-06-00_NA&si=0' for reading
[hls,applehttp @ 0x7ffff7f0d8a0] HLS request for url 'http://x-disney-datg-stgec.uplynk.com/80C078/ausc/slices/b45/21885d134fa441488df8e1d7acee8c66/b4594ce3d0b7447a9e43dbfa2128f450/J00000003.ts?pbs=1f50607d8d064a4eb22e72db53bff2f5&euid=C5B4DF9A-2D28-42FE-9841-4078C1C2A82C_000_0_001_lf_03-06-00_NA&si=0', offset 0, playlist 0
[hls,applehttp @ 0x7ffff7f0d8a0] Opening 'crypto+http://x-disney-datg-stgec.uplynk.com/80C078/ausc/slices/b45/21885d134fa441488df8e1d7acee8c66/b4594ce3d0b7447a9e43dbfa2128f450/J00000003.ts?pbs=1f50607d8d064a4eb22e72db53bff2f5&euid=C5B4DF9A-2D28-42FE-9841-4078C1C2A82C_000_0_001_lf_03-06-00_NA&si=0' for reading
[hls,applehttp @ 0x7ffff7f0d8a0] HLS request for url 'http://x-disney-datg-stgec.uplynk.com/80C078/ausc/slices/b45/21885d134fa441488df8e1d7acee8c66/b4594ce3d0b7447a9e43dbfa2128f450/J00000004.ts?pbs=1f50607d8d064a4eb22e72db53bff2f5&euid=C5B4DF9A-2D28-42FE-9841-4078C1C2A82C_000_0_001_lf_03-06-00_NA&si=0', offset 0, playlist 0
[hls,applehttp @ 0x7ffff7f0d8a0] Opening 'crypto+http://x-disney-datg-stgec.uplynk.com/80C078/ausc/slices/b45/21885d134fa441488df8e1d7acee8c66/b4594ce3d0b7447a9e43dbfa2128f450/J00000004.ts?pbs=1f50607d8d064a4eb22e72db53bff2f5&euid=C5B4DF9A-2D28-42FE-9841-4078C1C2A82C_000_0_001_lf_03-06-00_NA&si=0' for reading
[hls,applehttp @ 0x7ffff7f0d8a0] HLS request for url 'http://x-disney-datg-stgec.uplynk.com/80C078/ausc/slices/b45/21885d134fa441488df8e1d7acee8c66/b4594ce3d0b7447a9e43dbfa2128f450/J00000005.ts?pbs=1f50607d8d064a4eb22e72db53bff2f5&euid=C5B4DF9A-2D28-42FE-9841-4078C1C2A82C_000_0_001_lf_03-06-00_NA&si=0', offset 0, playlist 0
[hls,applehttp @ 0x7ffff7f0d8a0] Opening 'crypto+http://x-disney-datg-stgec.uplynk.com/80C078/ausc/slices/b45/21885d134fa441488df8e1d7acee8c66/b4594ce3d0b7447a9e43dbfa2128f450/J00000005.ts?pbs=1f50607d8d064a4eb22e72db53bff2f5&euid=C5B4DF9A-2D28-42FE-9841-4078C1C2A82C_000_0_001_lf_03-06-00_NA&si=0' for reading
[hls,applehttp @ 0x7ffff7f0d8a0] HLS request for url 'http://x-disney-datg-stgec.uplynk.com/80C078/ausc/slices/b45/21885d134fa441488df8e1d7acee8c66/b4594ce3d0b7447a9e43dbfa2128f450/J00000006.ts?pbs=1f50607d8d064a4eb22e72db53bff2f5&euid=C5B4DF9A-2D28-42FE-9841-4078C1C2A82C_000_0_001_lf_03-06-00_NA&si=0', offset 0, playlist 0
[hls,applehttp @ 0x7ffff7f0d8a0] Opening 'crypto+http://x-disney-datg-stgec.uplynk.com/80C078/ausc/slices/b45/21885d134fa441488df8e1d7acee8c66/b4594ce3d0b7447a9e43dbfa2128f450/J00000006.ts?pbs=1f50607d8d064a4eb22e72db53bff2f5&euid=C5B4DF9A-2D28-42FE-9841-4078C1C2A82C_000_0_001_lf_03-06-00_NA&si=0' for reading
[hls,applehttp @ 0x7ffff7f0d8a0] HLS request for url 'http://x-disney-datg-stgec.uplynk.com/80C078/ausc/slices/b45/21885d134fa441488df8e1d7acee8c66/b4594ce3d0b7447a9e43dbfa2128f450/J00000007.ts?pbs=1f50607d8d064a4eb22e72db53bff2f5&euid=C5B4DF9A-2D28-42FE-9841-4078C1C2A82C_000_0_001_lf_03-06-00_NA&si=0', offset 0, playlist 0
[hls,applehttp @ 0x7ffff7f0d8a0] Opening 'crypto+http://x-disney-datg-stgec.uplynk.com/80C078/ausc/slices/b45/21885d134fa441488df8e1d7acee8c66/b4594ce3d0b7447a9e43dbfa2128f450/J00000007.ts?pbs=1f50607d8d064a4eb22e72db53bff2f5&euid=C5B4DF9A-2D28-42FE-9841-4078C1C2A82C_000_0_001_lf_03-06-00_NA&si=0' for reading
^Cframe= 668 fps= 39 q=-1.0 Lsize= 11781kB time=00:00:28.03 bitrate=3442.8kbits/s speed=1.63x
video:11333kB audio:438kB subtitle:0kB other streams:0kB global headers:0kB muxing overhead: 0.086118%
Input file #0 (http://content-aeuf1.uplynk.com/b4594ce3d0b7447a9e43dbfa2128f450/j.m3u8?exp=1499075032&ct=a&oid=21885d134fa441488df8e1d7acee8c66&eid=10087805&iph=5f72106d5f10622d58c00a02db5f9015572ad88a12d556f4ede6918b51c26703&rays=jihgfedcb&euid=C5B4DF9A-2D28-42FE-9841-4078C1C2A82C_000_0_001_lf_03-06-00_NA&cdn=ec&stgcfg=datg&pp2ip=0&ddp=1&sig=c288780569bf6898bf9ad1d8d8246a929571bfde637f92ffdf44cacd49d2673d&pbs=1f50607d8d064a4eb22e72db53bff2f5):
Input stream #0:0 (audio): 658 packets read (448562 bytes);
Input stream #0:1 (video): 668 packets read (11604637 bytes);
Input stream #0:2 (data): 8 packets read (488 bytes);
Total: 1334 packets (12053687 bytes) demuxed
Output file #0 (file:S1 E1 - 13-VDKA3727119.mp4.part):
Output stream #0:0 (video): 668 packets muxed (11604637 bytes);
Output stream #0:1 (audio): 658 packets muxed (448562 bytes);
Total: 1326 packets (12053199 bytes) muxed
Exiting normally, received signal 2.
ERROR: Interrupted by user
```
</details>
<details>
<summary>Non-verbose log</summary>
```
1 nyuszika7h@panlyn ~ % youtube-dl --ignore-config 'http://watchdisneychannel.go.com/andi-mack/video/vdka3727119/01/01-13'
[Go] vdka3727119: Downloading JSON metadata
[Go] VDKA3727119: Downloading JSON metadata
[Go] VDKA3727119: Downloading m3u8 information
[download] Destination: S1 E1 - 13-VDKA3727119.mp4
ffmpeg version 3.3.2-1~16.04.york1 Copyright (c) 2000-2017 the FFmpeg developers
built with gcc 5.4.0 (Ubuntu 5.4.0-6ubuntu1~16.04.4) 20160609
configuration: --prefix=/usr --extra-version='1~16.04.york1' --toolchain=hardened --libdir=/usr/lib/x86_64-linux-gnu --incdir=/usr/include/x86_64-linux-gnu --enable-gpl --disable-stripping --enable-avresample --enable-avisynth --enable-gnutls --enable-ladspa --enable-libass --enable-libbluray --enable-libbs2b --enable-libcaca --enable-libcdio --enable-libflite --enable-libfontconfig --enable-libfreetype --enable-libfribidi --enable-libgme --enable-libgsm --enable-libmp3lame --enable-libopenjpeg --enable-libopenmpt --enable-libopus --enable-libpulse --enable-librubberband --enable-libshine --enable-libsnappy --enable-libsoxr --enable-libspeex --enable-libssh --enable-libtheora --enable-libtwolame --enable-libvorbis --enable-libvpx --enable-libwavpack --enable-libwebp --enable-libx265 --enable-libxvid --enable-libzmq --enable-libzvbi --enable-omx --enable-openal --enable-opengl --enable-sdl2 --enable-libdc1394 --enable-libiec61883 --enable-chromaprint --enable-frei0r --enable-libopencv --enable-libx264 --enable-shared
libavutil 55. 58.100 / 55. 58.100
libavcodec 57. 89.100 / 57. 89.100
libavformat 57. 71.100 / 57. 71.100
libavdevice 57. 6.100 / 57. 6.100
libavfilter 6. 82.100 / 6. 82.100
libavresample 3. 5. 0 / 3. 5. 0
libswscale 4. 6.100 / 4. 6.100
libswresample 2. 7.100 / 2. 7.100
libpostproc 54. 5.100 / 54. 5.100
[hls,applehttp @ 0x7fffd6b22860] Opening 'https://content-aeuf2.uplynk.com/check2?b=b4594ce3d0b7447a9e43dbfa2128f450&v=b4594ce3d0b7447a9e43dbfa2128f450&r=j&pbs=844db35aab0c4d348d668425d13c01e3' for reading
[hls,applehttp @ 0x7fffd6b22860] Opening 'crypto+http://x-disney-datg-stgec.uplynk.com/80C078/ausc/slices/b45/21885d134fa441488df8e1d7acee8c66/b4594ce3d0b7447a9e43dbfa2128f450/J00000000.ts?pbs=844db35aab0c4d348d668425d13c01e3&euid=F5CAA7F4-3C41-46F8-8287-675910000910_000_0_001_lf_03-06-00_NA&si=0' for reading
Input #0, hls,applehttp, from 'http://content-aeuf2.uplynk.com/b4594ce3d0b7447a9e43dbfa2128f450/j.m3u8?exp=1499075132&ct=a&oid=21885d134fa441488df8e1d7acee8c66&eid=10087805&iph=215aa527fb4ee1f791ba71f2064711593c5966885b28af1db6d3c23cac1552a1&rays=jihgfedcb&euid=F5CAA7F4-3C41-46F8-8287-675910000910_000_0_001_lf_03-06-00_NA&cdn=ec&stgcfg=datg&pp2ip=0&ddp=1&sig=6e5ee4834f8b18f48a7769e304e80a68146e217ddeb27ea5d4596dad1f726008&pbs=844db35aab0c4d348d668425d13c01e3':
Duration: 00:24:59.63, start: 0.000000, bitrate: 0 kb/s
Program 0
Metadata:
variant_bitrate : 0
Stream #0:0: Audio: aac (HE-AAC) ([15][0][0][0] / 0x000F), 48000 Hz, stereo, fltp
Metadata:
variant_bitrate : 0
Stream #0:1: Video: h264 (High) ([27][0][0][0] / 0x001B), yuv420p, 1280x720, 23.98 tbr, 90k tbn, 48 tbc
Metadata:
variant_bitrate : 0
Stream #0:2: Data: timed_id3 (ID3 / 0x20334449)
Metadata:
variant_bitrate : 0
Output #0, mp4, to 'file:S1 E1 - 13-VDKA3727119.mp4.part':
Metadata:
encoder : Lavf57.71.100
Stream #0:0: Video: h264 (High) ([33][0][0][0] / 0x0021), yuv420p, 1280x720, q=2-31, 23.98 tbr, 90k tbn, 90k tbc
Metadata:
variant_bitrate : 0
Stream #0:1: Audio: aac (HE-AAC) ([64][0][0][0] / 0x0040), 48000 Hz, stereo, fltp
Metadata:
variant_bitrate : 0
Stream mapping:
Stream #0:1 -> #0:0 (copy)
Stream #0:0 -> #0:1 (copy)
Press [q] to stop, [?] for help
[hls,applehttp @ 0x7fffd6b22860] Opening 'crypto+http://x-disney-datg-stgec.uplynk.com/80C078/ausc/slices/b45/21885d134fa441488df8e1d7acee8c66/b4594ce3d0b7447a9e43dbfa2128f450/J00000001.ts?pbs=844db35aab0c4d348d668425d13c01e3&euid=F5CAA7F4-3C41-46F8-8287-675910000910_000_0_001_lf_03-06-00_NA&si=0' for reading
[hls,applehttp @ 0x7fffd6b22860] Opening 'crypto+http://x-disney-datg-stgec.uplynk.com/80C078/ausc/slices/b45/21885d134fa441488df8e1d7acee8c66/b4594ce3d0b7447a9e43dbfa2128f450/J00000002.ts?pbs=844db35aab0c4d348d668425d13c01e3&euid=F5CAA7F4-3C41-46F8-8287-675910000910_000_0_001_lf_03-06-00_NA&si=0' for reading
[hls,applehttp @ 0x7fffd6b22860] Opening 'crypto+http://x-disney-datg-stgec.uplynk.com/80C078/ausc/slices/b45/21885d134fa441488df8e1d7acee8c66/b4594ce3d0b7447a9e43dbfa2128f450/J00000003.ts?pbs=844db35aab0c4d348d668425d13c01e3&euid=F5CAA7F4-3C41-46F8-8287-675910000910_000_0_001_lf_03-06-00_NA&si=0' for reading
[hls,applehttp @ 0x7fffd6b22860] Opening 'crypto+http://x-disney-datg-stgec.uplynk.com/80C078/ausc/slices/b45/21885d134fa441488df8e1d7acee8c66/b4594ce3d0b7447a9e43dbfa2128f450/J00000004.ts?pbs=844db35aab0c4d348d668425d13c01e3&euid=F5CAA7F4-3C41-46F8-8287-675910000910_000_0_001_lf_03-06-00_NA&si=0' for reading
[hls,applehttp @ 0x7fffd6b22860] Opening 'crypto+http://x-disney-datg-stgec.uplynk.com/80C078/ausc/slices/b45/21885d134fa441488df8e1d7acee8c66/b4594ce3d0b7447a9e43dbfa2128f450/J00000005.ts?pbs=844db35aab0c4d348d668425d13c01e3&euid=F5CAA7F4-3C41-46F8-8287-675910000910_000_0_001_lf_03-06-00_NA&si=0' for reading
^C^C
ERROR: Interrupted by user
```
</details>
---
### Description of your *issue*, suggested solution and other information
The FFmpeg console output is too verbose when downloading from go.com (Watch Disney) even when `-v` is not used. There are no problems with the download though, it completes successfully.
|
external-bugs
|
low
|
Critical
|
240,220,245 |
youtube-dl
|
[bbc] BBC Sport streams throw CertificateError/RegexNotFoundError
|
### Make sure you are using the *latest* version: run `youtube-dl --version` and ensure your version is *2017.07.02*. If it's not read [this FAQ entry](https://github.com/rg3/youtube-dl/blob/master/README.md#how-do-i-update-youtube-dl) and update. Issues with outdated version will be rejected.
- [x] I've **verified** and **I assure** that I'm running youtube-dl **2017.07.02**
### Before submitting an *issue* make sure you have:
- [x] At least skimmed through [README](https://github.com/rg3/youtube-dl/blob/master/README.md) and **most notably** [FAQ](https://github.com/rg3/youtube-dl#faq) and [BUGS](https://github.com/rg3/youtube-dl#bugs) sections
- [x] [Searched](https://github.com/rg3/youtube-dl/search?type=Issues) the bugtracker for similar issues including closed ones
### What is the purpose of your *issue*?
- [x] Bug report (encountered problems with youtube-dl)
- [ ] Site support request (request for adding support for a new site)
- [ ] Feature request (request for a new functionality)
- [ ] Question
- [ ] Other
### If the purpose of this *issue* is a *bug report*, *site support request* or you are not completely sure provide the full verbose output as follows:
Add `-v` flag to **your command line** you run youtube-dl with, copy the **whole** output and insert it here. It should look similar to one below (replace it with **your** log inserted between triple ```):
```
λ youtube-dl -o - -v http://www.bbc.co.uk/sport/live/tennis/39942487 | "C:\Program Files\VideoLAN\VLC\vlc.exe" -
[debug] System config: []
[debug] User config: []
[debug] Custom config: []
[debug] Command-line args: ['-o', '-', '-v', 'http://www.bbc.co.uk/sport/live/tennis/39942487']
[debug] Encodings: locale cp1252, fs utf-8, out cp1252, pref cp1252
[debug] youtube-dl version 2017.07.02
[debug] Python version 3.6.0 - Windows-10-10.0.15063-SP0
[debug] exe versions: ffmpeg N-85588-g6108805, ffprobe N-85588-g6108805
[debug] Proxy map: {}
[bbc] 39942487: Downloading webpage
[bbc] p055ysdk: Downloading media selection XML
[bbc] p055ysdk: Downloading m3u8 information
[bbc] p055ysdk: Downloading m3u8 information
[bbc] p055ysdk: Downloading m3u8 information
[bbc] p055ysdk: Downloading m3u8 information
[debug] Invoking downloader on 'https://ve-hls-uk-live.bbcfmt.hs.llnwd.net/pool_47/live/uk/sport_stream_15/sport_stream_15.isml/sport_stream_15-pa4%3d128000-video%3d5070016.m3u8'
[hlsnative] Downloading m3u8 manifest
Traceback (most recent call last):
File "c:\dev\python36\lib\runpy.py", line 193, in _run_module_as_main
"__main__", mod_spec)
File "c:\dev\python36\lib\runpy.py", line 85, in _run_code
exec(code, run_globals)
File "C:\Dev\Python36\Scripts\youtube-dl.exe\__main__.py", line 9, in <module>
File "c:\dev\python36\lib\site-packages\youtube_dl\__init__.py", line 465, in main
_real_main(argv)
File "c:\dev\python36\lib\site-packages\youtube_dl\__init__.py", line 455, in _real_main
retcode = ydl.download(all_urls)
File "c:\dev\python36\lib\site-packages\youtube_dl\YoutubeDL.py", line 1927, in download
url, force_generic_extractor=self.params.get('force_generic_extractor', False))
File "c:\dev\python36\lib\site-packages\youtube_dl\YoutubeDL.py", line 773, in extract_info
return self.process_ie_result(ie_result, download, extra_info)
File "c:\dev\python36\lib\site-packages\youtube_dl\YoutubeDL.py", line 827, in process_ie_result
return self.process_video_result(ie_result, download=download)
File "c:\dev\python36\lib\site-packages\youtube_dl\YoutubeDL.py", line 1570, in process_video_result
self.process_info(new_info)
File "c:\dev\python36\lib\site-packages\youtube_dl\YoutubeDL.py", line 1834, in process_info
success = dl(filename, info_dict)
File "c:\dev\python36\lib\site-packages\youtube_dl\YoutubeDL.py", line 1776, in dl
return fd.download(name, info)
File "c:\dev\python36\lib\site-packages\youtube_dl\downloader\common.py", line 361, in download
return self.real_download(filename, info_dict)
File "c:\dev\python36\lib\site-packages\youtube_dl\downloader\hls.py", line 62, in real_download
manifest = self.ydl.urlopen(self._prepare_url(info_dict, man_url)).read()
File "c:\dev\python36\lib\site-packages\youtube_dl\YoutubeDL.py", line 2137, in urlopen
return self._opener.open(req, timeout=self._socket_timeout)
File "c:\dev\python36\lib\urllib\request.py", line 532, in open
response = meth(req, response)
File "c:\dev\python36\lib\urllib\request.py", line 642, in http_response
'http', request, response, code, msg, hdrs)
File "c:\dev\python36\lib\urllib\request.py", line 564, in error
result = self._call_chain(*args)
File "c:\dev\python36\lib\urllib\request.py", line 504, in _call_chain
result = func(*args)
File "c:\dev\python36\lib\urllib\request.py", line 756, in http_error_302
return self.parent.open(new, timeout=req.timeout)
File "c:\dev\python36\lib\urllib\request.py", line 526, in open
response = self._open(req, data)
File "c:\dev\python36\lib\urllib\request.py", line 544, in _open
'_open', req)
File "c:\dev\python36\lib\urllib\request.py", line 504, in _call_chain
result = func(*args)
File "c:\dev\python36\lib\site-packages\youtube_dl\utils.py", line 1086, in https_open
req, **kwargs)
File "c:\dev\python36\lib\urllib\request.py", line 1318, in do_open
encode_chunked=req.has_header('Transfer-encoding'))
File "c:\dev\python36\lib\http\client.py", line 1239, in request
self._send_request(method, url, body, headers, encode_chunked)
File "c:\dev\python36\lib\http\client.py", line 1285, in _send_request
self.endheaders(body, encode_chunked=encode_chunked)
File "c:\dev\python36\lib\http\client.py", line 1234, in endheaders
self._send_output(message_body, encode_chunked=encode_chunked)
File "c:\dev\python36\lib\http\client.py", line 1026, in _send_output
self.send(msg)
File "c:\dev\python36\lib\http\client.py", line 964, in send
self.connect()
File "c:\dev\python36\lib\http\client.py", line 1400, in connect
server_hostname=server_hostname)
File "c:\dev\python36\lib\ssl.py", line 401, in wrap_socket
_context=self, _session=session)
File "c:\dev\python36\lib\ssl.py", line 808, in __init__
self.do_handshake()
File "c:\dev\python36\lib\ssl.py", line 1061, in do_handshake
self._sslobj.do_handshake()
File "c:\dev\python36\lib\ssl.py", line 688, in do_handshake
match_hostname(self.getpeercert(), self.server_hostname)
File "c:\dev\python36\lib\ssl.py", line 321, in match_hostname
% (hostname, ', '.join(map(repr, dnsnames))))
ssl.CertificateError: hostname 'bbcfmt-ic-2d4c8800-14b7be-vehlsuklive.s.loris.llnwd.net' doesn't match either of '*.akamaihd.net', '*.akamaihd-staging.net', '*.akamaized-staging.net', '*.akamaized.net', 'a248.e.akamai.net'
```
```
λ youtube-dl -o - -v --no-check-certificate http://www.bbc.co.uk/sport/live/tennis/39942487 | "C:\Program Files\VideoLAN\VLC\vlc.exe" -
[debug] System config: []
[debug] User config: []
[debug] Custom config: []
[debug] Command-line args: ['-o', '-', '-v', '--no-check-certificate', 'http://www.bbc.co.uk/sport/live/tennis/39942487']
[debug] Encodings: locale cp1252, fs utf-8, out cp1252, pref cp1252
[debug] youtube-dl version 2017.07.02
[debug] Python version 3.6.0 - Windows-10-10.0.15063-SP0
[debug] exe versions: ffmpeg N-85588-g6108805, ffprobe N-85588-g6108805
[debug] Proxy map: {}
[bbc] 39942487: Downloading webpage
ERROR: Unable to extract playlist data; please report this issue on https://yt-dl.org/bug . Make sure you are using the latest version; see https://yt-dl.org/update on how to update. Be sure to call youtube-dl with the --verbose flag and include its complete output.
Traceback (most recent call last):
File "c:\dev\python36\lib\site-packages\youtube_dl\YoutubeDL.py", line 762, in extract_info
ie_result = ie.extract(url)
File "c:\dev\python36\lib\site-packages\youtube_dl\extractor\common.py", line 433, in extract
ie_result = self._real_extract(url)
File "c:\dev\python36\lib\site-packages\youtube_dl\extractor\bbc.py", line 1033, in _real_extract
webpage, 'playlist data'),
File "c:\dev\python36\lib\site-packages\youtube_dl\extractor\common.py", line 782, in _search_regex
raise RegexNotFoundError('Unable to extract %s' % _name)
youtube_dl.utils.RegexNotFoundError: Unable to extract playlist data; please report this issue on https://yt-dl.org/bug . Make sure you are using the latest version; see https://yt-dl.org/update on how to update. Be sure to call youtube-dl with the --verbose flag and include its complete output.
```
Using youtube-dl to watch Wimbledon streams on BBC Sport does not work for me at the moment. Apparently some certificate seems to be invalid (see 1st log) - not sure if this is caused by youtube-dl, but it works in my browser. I then tried to skip the certificate check, but ytdl throws a RegexNotFoundError (see 2nd log). Probably something was changed on the BBC website?
|
geo-restricted
|
low
|
Critical
|
240,227,707 |
vscode
|
Language services should be able to provide debug console IntelliSense
|
### Scenario
Currently, debug adapters can provide IntelliSense in the console. This is great for untyped or weakly typed languages like JavaScript/TypeScript. But for statically typed languages, such as C#, the best way to do this is to let the regular language service provide IntelliSense just like in the editor.
### Basic proposal
In the language service registration for an extension, a language service can indicate that it supports debug console IntelliSense. If the language of the document of the current stack frame opts into this, then instead of going down the path where completion is requested from debug adapter, it is instead requested from the language service. VS Code would need to provide the location of the active stack frame in the same way that regular intellisense works. Then it would need to provide the text and position of the line being edited in the debug console.
Assuming that VS Code is eventually interested in supporting this, https://github.com/OmniSharp/omnisharp-vscode/issues/1609 tracks the work in the C# extension to support this.
|
feature-request,debug
|
low
|
Critical
|
240,228,385 |
rust
|
rustdoc: it would be nice to have a way to extract the doctest source without building it
|
It would be nice to have a way to extract the doctest sources into a file without compiling or running the test. I would like to integrate doctests into our current build system, and it looks like that would be easiest if I could extract the doc tests as source, then just build them as normal unit tests.
|
T-rustdoc,C-feature-request,A-doctests
|
low
|
Minor
|
240,229,166 |
rust
|
rustdoc: add the ability to build a doctest executable without running it
|
For flexibility of integration with other testing frameworks/infrastructure, it would be nice if rustdoc had a mechanism to build doctest executables without running them, so that they can be later run within a separate test coordination framework.
|
T-rustdoc,C-feature-request,A-doctests
|
low
|
Minor
|
240,229,204 |
rust
|
rustdoc: allow full set of compiler options to be specified
|
`rustdoc` doesn't accept the full set of compiler options (in particular `-C`), so it isn't possible to build doctests in the same way as crates, binaries and normal unit tests. The ideal would be to just allow the test source to be extracted (#43029), so that building the doctest is identical to other code.
Failing that, some systematic way of converting a rustc compiler command line to a rustdoc one for building doctests would be OK.
(Filed separately, the ability to just build a test without running it: #43030)
|
T-rustdoc,C-feature-request,A-doctests
|
low
|
Minor
|
240,241,053 |
rust
|
Tracking issue for RFC 1985: Tiered browser support
|
[RFC](https://github.com/rust-lang/rfcs/pull/1985)
- [ ] Document our tiered browser support policy as agreed upon in the RFC
- [ ] In [the forge](https://github.com/rust-lang-nursery/rust-forge) - should "browser support" get a different page or should it be added to the [platform support page](https://forge.rust-lang.org/platform-support.html)?
- [ ] In crates.io/cargo's docs ([faq](http://doc.crates.io/faq.html)? [policies](http://doc.crates.io/policies.html)? [contributing.md](https://github.com/rust-lang/crates.io/blob/master/docs/CONTRIBUTING.md)? all of the above? somewhere else?)
- [ ] Somewhere for rustdoc (this is sort of in flux right now)
- [ ] [rust-lang.org's README](https://github.com/rust-lang/rust-www/)
- [ ] [blog.rust-lang.org's README](https://github.com/rust-lang/blog.rust-lang.org)
- [ ] the playground's README (this is sort of in flux right now)
- [ ] [thanks.rust-lang.org's README](https://github.com/rust-lang-nursery/thanks)
- [ ] Should we add a note about this to these repos' issue templates?
- [ ] Add automated testing using BrowserStack to start testing some functionality in each of the the supported browsers. The following sites are complex enough that they deserve testing IMO, the rest are mostly static content and we can rely on bug reports:
- [ ] For crates.io
- [ ] For rustdoc
- [ ] For the playground
- [ ] Close any issues in repos that only apply to browsers that we've decided are unsupported
|
B-RFC-approved,T-infra,C-tracking-issue
|
low
|
Critical
|
240,247,079 |
electron
|
Make desktop notification clickable on Linux
|
This is a feature request. As of now notifications are clickable on macOS and Windows but not on Linux.
|
enhancement :sparkles:,platform/linux
|
low
|
Major
|
240,250,850 |
go
|
x/crypto/bcrypt: API to check hash format
|
golang.org/x/crypto/bcrypt [1] does not provide an official API to verify that the hash format is good one. The current sources indicates [2] that one can use bcrypt.Cost() as that parses and verifies the whole hash string. But the documentation does not reflect that a successful return from the function indicates a valid hash. Also the documentation implies that bcrypt.ErrHashTooShort can only be returned from CompareHashAndPassword when the Cost function can also return it.
It would be nice to have an explicit API to check hash format and perhaps check that it was created by older version so a password change can be scheduled in such case.
[1] - https://godoc.org/golang.org/x/crypto/bcrypt
[2] - https://github.com/golang/crypto/blob/6c586e17d90a7d08bbbc4069984180dce3b04117/bcrypt/bcrypt.go#L118
|
NeedsInvestigation
|
low
|
Minor
|
240,279,919 |
opencv
|
cvStartWindowThread implementations are still missing for multiple ui frameworks
|
##### System information (version)
- OpenCV => 3.1
- Operating System / Platform => Ubuntu/Mac
- Compiler => gcc/clang
##### Detailed description
cvStartWindowThread is only implemented only for gtk. The implementations for cocoa, qt and winrt, carbon, etc are still missing.
|
feature,category: highgui-gui
|
low
|
Minor
|
240,309,906 |
TypeScript
|
(Proposal) syntax for contextual typing of a function declaration
|
Currently, it is possible to give a function a contextual type if it is a function expression. This is often used by callback types, and by certain interface types that add properties, or specify the parameter types / return type of a function.
A common use, in the declaration case, is `React.SFC`:
```tsx
interface Props {
propName?: string
}
const Component: React.SFC<Props> = props => <div>{props.propName!}</div>
Component.defaultProps = { propName: 'propName' }
```
In the snippet above, `props` is contextually typed, and the function is only allowed to return a `React.Element` or `null`. The function object also has several keys added to it, most notably `defaultProps`, `displayName` and `propTypes`.
However, there is no code reason for why `Component` needs to be a function expression. It could as well be a function declaration. However, making it a function declaration prevents it from being typed as `React.SFC`.
Not being able to give it a type requires that all parameters have the types manually written, and also prevents attaching the extra property keys such as `defaultProps`. It will also not validate the function's return type -- you will instead get an error when you attempt to use the function if it happens to be incompatible.
The idea here is to have a way to tell the compiler what the "contextual type" of a function declaration should be.
One possible syntax could be appropriating the `implements` keyword used for `class`:
```ts
interface Props {
propName?: string
}
Component.defaultProps = { propName: 'propName' }
function Component (props) implements React.SFC<Props> {
return <div>{props.propName!}</div>
}
```
This would make it so `Component` uses `React.SFC<Props>` type as its type, similar to if it was a function expression that has a context given to it. This should have no effect on code emit and can simply be stripped on emit; similarly to the `class` `implements` clause.
In more generic terms,
```ts
function FunctionName (...ArgumentList) implements TypeExpression FunctionBody
```
should behave similarly to
```ts
var FunctionName: TypeExpression = function (...ArgumentList) FunctionBody
```
but be an actual hoisted function declaration.
---
Having a way of specifying the type like this also could make for easier refactoring of callbacks -- when extracting a callback from being given as an expression to being a named declaration, you often have to go to the type declaration and copy&paste the signature types to the extracted declaration.
With something like the proposed `implements`, you will still need to add a type, but it will only be the original context type; which then removes the need of explicitly giving types to each argument and the return type.
|
Suggestion,In Discussion
|
low
|
Critical
|
240,355,800 |
youtube-dl
|
Crash on one (out of 24) videos on Sofia the first
|
## Please follow the guide below
- You will be asked some questions and requested to provide some information, please read them **carefully** and answer honestly
- Put an `x` into all the boxes [ ] relevant to your *issue* (like that [x])
- Use *Preview* tab to see how your issue will actually look like
---
### Make sure you are using the *latest* version: run `youtube-dl --version` and ensure your version is *2017.07.02*. If it's not read [this FAQ entry](https://github.com/rg3/youtube-dl/blob/master/README.md#how-do-i-update-youtube-dl) and update. Issues with outdated version will be rejected.
- [ x] I've **verified** and **I assure** that I'm running youtube-dl **2017.07.02**
### Before submitting an *issue* make sure you have:
- [x ] At least skimmed through [README](https://github.com/rg3/youtube-dl/blob/master/README.md) and **most notably** [FAQ](https://github.com/rg3/youtube-dl#faq) and [BUGS](https://github.com/rg3/youtube-dl#bugs) sections
- [x ] [Searched](https://github.com/rg3/youtube-dl/search?type=Issues) the bugtracker for similar issues including closed ones
### What is the purpose of your *issue*?
- [x ] Bug report (encountered problems with youtube-dl)
- [ ] Site support request (request for adding support for a new site)
- [ ] Feature request (request for a new functionality)
- [ ] Question
- [ ] Other
---
### The following sections concretize particular purposed issues, you can erase any section (the contents between triple ---) not applicable to your *issue*
---
### If the purpose of this *issue* is a *bug report*, *site support request* or you are not completely sure provide the full verbose output as follows:
Add `-v` flag to **your command line** you run youtube-dl with, copy the **whole** output and insert it here. It should look similar to one below (replace it with **your** log inserted between triple ```):
```
keybounceMBP:Disney michael$ ./yt-dl -v http://watchdisneyjunior.go.com/sofia-the-first
[debug] System config: []
[debug] User config: ['-k', '-o', '%(title)s.%(ext)s', '-f', '\nbest[ext=mp4][height>431][height<=576]/\nbestvideo[ext=mp4][height=480]+bestaudio[ext=m4a]/\nbest[ext=mp4][height>340][height<=431]/\nbestvideo[ext=mp4][height>360][height<=576]+bestaudio/\nbest[height>340][height<=576]/\nbestvideo[height>360][height<=576]+bestaudio/\nbestvideo[height=360]+bestaudio/\nbest[ext=mp4][height>=280][height<=360]/\nbest[height<=576]/\nworst', '--ap-mso', 'Dish', '--ap-username', 'PRIVATE', '--ap-password', 'PRIVATE', '--write-sub', '--write-auto-sub', '--sub-lang', 'en,enUS,en-us', '--sub-format', 'ass/srt/best', '--convert-subs', 'ass', '--embed-subs', '--mark-watched', '--download-archive', 'downloaded-videos.txt']
[debug] Custom config: []
[debug] Command-line args: ['-v', '--hls-prefer-native', '-o', '%(series)s/s%(season_number)02d-e%(episode_number)02d-%(title)s.%(ext)s', '-v', 'http://watchdisneyjunior.go.com/sofia-the-first']
[debug] Encodings: locale UTF-8, fs utf-8, out UTF-8, pref UTF-8
[debug] youtube-dl version 2017.07.02
[debug] Python version 3.6.1 - Darwin-13.4.0-x86_64-i386-64bit
[debug] exe versions: ffmpeg 3.2.4, ffprobe 3.2.4, rtmpdump 2.4
[debug] Proxy map: {}
[Go] sofia-the-first: Downloading webpage
[Go] SH55246331: Downloading JSON metadata
[download] Downloading playlist: Sofia the First
[Go] playlist Sofia the First: Collected 24 video ids (downloading 24 of them)
[download] Downloading video 1 of 24
[download] Music Video: True Sisters has already been recorded in archive
[download] Downloading video 2 of 24
[download] Music Video: Blue Ribbon Bunny has already been recorded in archive
[download] Downloading video 3 of 24
[download] Rise and Shine (Ariel Winter) has already been recorded in archive
[download] Downloading video 4 of 24
[download] Dare to Risk It All has already been recorded in archive
[download] Downloading video 5 of 24
[download] Bigger is Better has already been recorded in archive
[download] Downloading video 6 of 24
[download] Make It Right has already been recorded in archive
[download] Downloading video 7 of 24
[download] Small New World has already been recorded in archive
[download] Downloading video 8 of 24
[download] Stronger Than You Know has already been recorded in archive
[download] Downloading video 9 of 24
[download] Theme Song has already been recorded in archive
[download] Downloading video 10 of 24
[download] Theme Song has already been recorded in archive
[download] Downloading video 11 of 24
[download] A Knight Such As I has already been recorded in archive
[download] Downloading video 12 of 24
[download] This Panda Just Wants to Dance has already been recorded in archive
[download] Downloading video 13 of 24
[download] Enchancia Fashion Insider with Tim Gunn has already been recorded in archive
[download] Downloading video 14 of 24
[download] The Broomstick Dance has already been recorded in archive
[download] Downloading video 15 of 24
[download] Ghostly Gala has already been recorded in archive
[download] Downloading video 16 of 24
[download] S3 E24: Royal Vacation has already been recorded in archive
[download] Downloading video 17 of 24
[download] S4 E1: Day of the Sorcerers has already been recorded in archive
[download] Downloading video 18 of 24
[download] S4 E2: The Secret Library: Tale of the Eternal Torch has already been recorded in archive
[download] Downloading video 19 of 24
[download] S4 E3: The Crown of Blossoms has already been recorded in archive
[download] Downloading video 20 of 24
[download] S4 E4: Pin the Blame on the Genie has already been recorded in archive
[download] Downloading video 21 of 24
[download] S4 E5: The Mystic Isles has already been recorded in archive
[download] Downloading video 22 of 24
[download] S4 E6: The Mystic Isles: The Princess and the Protector has already been recorded in archive
[download] Downloading video 23 of 24
[download] Let's Play Sofia the First! has already been recorded in archive
[download] Downloading video 24 of 24
[Go] vdka3952398: Downloading JSON metadata
[Go] VDKA3952398: Retrieving Media Token
[Go] VDKA3952398: Downloading JSON metadata
[Go] VDKA3952398: Downloading m3u8 information
WARNING: en subtitles not available for VDKA3952398
WARNING: enUS subtitles not available for VDKA3952398
[info] Writing video subtitles to: Sofia the First/s01-e123-Two Episodes.en-us.ttml
[debug] Invoking downloader on 'http://content-ause3.uplynk.com/d9082eb62eb34819b9e0019b7e04d687/f.m3u8?exp=1499158782&ct=a&oid=21885d134fa441488df8e1d7acee8c66&eid=10097567&iph=8cbc60807f773b928fa894609146e614c72cd33d54465e9e70dd7dfcdcea8c3f&rays=jihgfedcb&euid=CA3F7063-D15B-4B9E-8AE8-7CE99D41EE33_000_1_001_mp_04-06-00_NA&cdn=ec&stgcfg=datg&pp2ip=0&ddp=1&sig=ace269199a76369f87f3ffe3ad1ee503d19606b8cfb1c32ad2c818d9d00fb7b6&pbs=b109ec45d5af413ebfbfcd1e6538a89c'
[hlsnative] Downloading m3u8 manifest
[hlsnative] Total fragments: 1297
[download] Destination: Sofia the First/s01-e123-Two Episodes.mp4
[download] 44.7% of ~742.71MiB at Unknown speed ETA Unknown ETATraceback (most recent call last):
File "/opt/local/Library/Frameworks/Python.framework/Versions/3.6/lib/python3.6/runpy.py", line 193, in _run_module_as_main
"__main__", mod_spec)
File "/opt/local/Library/Frameworks/Python.framework/Versions/3.6/lib/python3.6/runpy.py", line 85, in _run_code
exec(code, run_globals)
File "/Users/michael/bin/youtube-dl/__main__.py", line 19, in <module>
File "/Users/michael/bin/youtube-dl/youtube_dl/__init__.py", line 465, in main
File "/Users/michael/bin/youtube-dl/youtube_dl/__init__.py", line 455, in _real_main
File "/Users/michael/bin/youtube-dl/youtube_dl/YoutubeDL.py", line 1927, in download
File "/Users/michael/bin/youtube-dl/youtube_dl/YoutubeDL.py", line 773, in extract_info
File "/Users/michael/bin/youtube-dl/youtube_dl/YoutubeDL.py", line 964, in process_ie_result
File "/Users/michael/bin/youtube-dl/youtube_dl/YoutubeDL.py", line 834, in process_ie_result
File "/Users/michael/bin/youtube-dl/youtube_dl/YoutubeDL.py", line 773, in extract_info
File "/Users/michael/bin/youtube-dl/youtube_dl/YoutubeDL.py", line 827, in process_ie_result
File "/Users/michael/bin/youtube-dl/youtube_dl/YoutubeDL.py", line 1570, in process_video_result
File "/Users/michael/bin/youtube-dl/youtube_dl/YoutubeDL.py", line 1834, in process_info
File "/Users/michael/bin/youtube-dl/youtube_dl/YoutubeDL.py", line 1776, in dl
File "/Users/michael/bin/youtube-dl/youtube_dl/downloader/common.py", line 361, in download
File "/Users/michael/bin/youtube-dl/youtube_dl/downloader/hls.py", line 149, in real_download
File "/opt/local/Library/Frameworks/Python.framework/Versions/3.6/lib/python3.6/site-packages/Crypto/Cipher/blockalgo.py", line 295, in decrypt
return self._cipher.decrypt(ciphertext)
ValueError: Input strings must be a multiple of 16 in length
keybounceMBP:Disney michael$
```
|
tv-provider-account-needed
|
low
|
Critical
|
240,380,828 |
kubernetes
|
StatefulSet numbering offset
|
/kind feature
Hi
it would be nice if StatefulSets could set an offset to their numbering - a lot of applications often start counting from 1 making it a bit messy translating from 0 to 1 between applications ("app part 1 runs on app-0.app.default.svc.cluster.local" etc). Or when having one load spread across multiple clusters - one going 0-20 and second going 21-40 for example to spread failover capabilities or just spread the load.
Ashley
|
area/usability,kind/feature,area/stateful-apps,sig/apps,lifecycle/frozen
|
low
|
Major
|
240,409,320 |
rust
|
Possibly spurious overlapping impl (even with specialization)
|
The following code leads to an "overlapping impl" error despite the fact that the `bool` impl is clearly more specific (totally concrete).
```rust
#![feature(specialization)]
trait Encodable {
fn encode() {}
}
trait Decodable {
fn decode() {}
}
impl Encodable for bool {
fn encode() {}
}
impl Decodable for bool {
fn decode() {}
}
impl<D> Encodable for D where for<'a> &'a D: AsRef<[u8]> {
default fn encode() {}
}
impl<T: for<'a> From<&'a [u8]>> Decodable for T {
default fn decode() {}
}
```
|
A-specialization,C-bug,F-specialization
|
low
|
Critical
|
240,418,044 |
rust
|
Weird interaction with projections in trait bounds
|
Reproduction case here: https://is.gd/KJTI1j.
This code should compile fine, as it's simply stating a constraint and then something attempting to use it. Regardless of whether the projection is unified with the real type or not, this code should be able to compile. Replacing the projection on line 22 with a concrete type will cause the issue to go away.
|
A-type-system,T-compiler,C-bug,T-types
|
low
|
Minor
|
240,452,711 |
go
|
image/png: Decode failing on bitmap
|
Please answer these questions before submitting your issue. Thanks!
### What version of Go are you using (`go version`)?
go version go1.8.3 windows/amd64
### What operating system and processor architecture are you using (`go env`)?
set GOARCH=amd64
set GOOS=windows
### What did you do?
See https://play.golang.org/p/-1XYQAzugF
Trying to decode a bitmap png file which both chrome and photoshop can open without errors.
### What did you expect to see?
A decoded png image, since no bitmap format is defined mapped to a 8-bit file?
### What did you see instead?
png: invalid format: too much pixel data
To my understanding this is a valid png file and according to the spec a decoder should be able to read all valid png files though it may not be able to encode them.
|
NeedsDecision
|
low
|
Critical
|
240,463,442 |
neovim
|
API/UI: externalize 'relativenumber'
|
- `nvim --version`: VIM v0.2.1-272-gfe476ed (on https://github.com/neovim/neovim/pull/6816 to fix scrolling problems on master), but I do not remember not having this problem
- Vim (version: ) behaves differently? could not check, no vim available
- Operating system/version: Kubuntu 16.10
- Terminal name/version: Alacritty / rxvt-unicode / konsole
- `$TERM`: xterm-256color
### Actual behaviour
When a big file is opened and modified for a long time, the scrolling gets laggier and laggier. I tried to narrow it down to a plugin / action (over the course of a few months), but in the end what "solves" it is switching to `norelativenumber`.
I tried to profile the script times (with `profile func / file *`) but nothing seems out of place. Syntax parsing is not especially slow. There aren't any autocommands on `CursorMoved` but for [neomake](https://github.com/neomake/neomake), [airline](https://github.com/vim-airline/vim-airline) and [syntastic](https://github.com/vim-syntastic/syntastic), but disabling those plugins did not help.
To sum it up, I open some big file and work on it for a long time, and scrolling is getting laggier and laggier over time. I noticed that the more folds are opened, the laggier it gets. Also, it is "per buffer" (a newly opened buffer will not have the problem unless I spend a long time on it). Finally, `set norelativenumber` instantly solves the problem. Setting `relativenumber` back will make it as laggy as before I disabled it.
Once the lagging is noticeable, I tried switching the filetype to no avail (to remove the possibility of bad syntax file), disabling all plugins that can be dynamically disabled, but in the end `set norelativedelta` is the trigger.
Also (to be clear), the "lag" is actually my CPU running 100%, and it also happens in insert mode, though the easiest and most annoying proof is scrolling.
### Expected behaviour
Not such a huge performance difference between `relativenumber` and `norelativenumber`
### Steps to reproduce using `nvim -u NORC`
**Edit :** I understand there may not be enough information to solve this, and I can live without `relativenumber`. I will try to narrow it down by trimming my init.vim but this may take some days / weeks
**Edit 2 :** Finally narrowed down the cause of the problem, it was the [indentLine](https://github.com/Yggdroot/indentLine) (detailed report [here](https://github.com/Yggdroot/indentLine/issues/58#issuecomment-314718710)). I do not really understand how I missed it since it never appeared in any report (syntime / profile), but removing it totally solved it (though there still is a difference between using `set relativenumber` and `set norelativenumber`)
|
performance,api,ui,ui-extensibility,column
|
medium
|
Major
|
240,509,897 |
go
|
x/mobile: build error when compiling with openal
|
### What version of Go are you using (`go version`)?
go version go1.8.1 darwin/amd64
gomobile version +44a54e9 Wed May 24 10:27:38 2017 +0000 (android,ios); androidSDK=
### What operating system and processor architecture are you using (`go env`)?
MacOS10.12.1
### What did you do?
gomobile init -ndk /Users/bluepants/Documents/adt-bundle-mac-x86_64-20131030/sdk/ndk-bundle -openal "/Users/bluepants/Downloads/openal-soft-1.18.0"
gomobile build
### What did you expect to see?
SUCCESS
### What did you see instead?
gomobile: the Android requires the golang.org/x/mobile/exp/audio/al, but the OpenAL libraries was not found. Please run gomobile init with the -openal flag pointing to an OpenAL source directory.
Is somewhere I did wrong?Why did I can't do it right?Please help me.
|
mobile
|
low
|
Critical
|
240,511,034 |
godot
|
get_tree().quit() not working when running in Remote File System mode
|
**Operating system or device - Godot version:**
macOS, Windows, iOS - Godot 2.1.3
**Issue description:**
Running the game in Windows and iOS with the argument "-rfs IP" and calling get_tree().quit() causes the executable to become unresponsive.
Running the game from the editor works as expected
|
bug,platform:ios,topic:core,topic:porting
|
low
|
Major
|
240,547,125 |
TypeScript
|
Allow extending multiple interfaces with different, but compatible types
|
```ts
interface Change {
uid: string;
type: string;
}
interface SomeChangeExtension {
type: 'some';
foo: number;
}
interface SomeChange extends Change, SomeChangeExtension { }
```
In this example, I was expecting `SomeChange` to have a type equivalent to:
```ts
interface SomeChange {
uid: string;
type: 'some';
foo: number;
}
```
But it would result in error:
```
Interface 'SomeChange' cannot simultaneously extend types 'Change' and 'SomeChangeExtension'.
Named property 'type' of types 'Change' and 'SomeChangeExtension' are not identical.
```
In this case, `'some'` is compatible with `string` if the order of interfaces being extended is respected.
The reason why I want this to be allowed is that, I need to maintain multiple interfaces of the same kind of change during different stages: **raw change**, **change**, **broadcast change**. And having to duplicate part of the "extension" on every of them doesn't look good.
|
Suggestion,Needs Proposal
|
high
|
Critical
|
240,586,407 |
go
|
math/big: big.Int String conversion is slow
|
(Forked out from #11068 which is about slow `big.Float` printing but will likely be fixed by not converting in full precision before printing).
The following program:
```
package main
import (
"fmt"
"math/big"
)
func main() {
n := new(big.Int)
n.Exp(big.NewInt(2), big.NewInt(5000000), nil)
fmt.Println(n)
}
```
which computes `2**5000000` and prints it, takes about 7 seconds on my machine to print the 1505151 characters-long answer.
```
$ time ./test > /dev/null
real 0m6.847s
user 0m6.848s
sys 0m0.004s
```
Almost all the time is spent generating the `String` representation of `n`. For comparison, the following equivalent `gmp` program:
```
#include <gmp.h>
int main(void)
{
mpz_t n, b;
mpz_inits(n, b);
mpz_set_ui(b, 2);
mpz_pow_ui(n, b, 5000000);
gmp_printf("%Zd\n", n);
}
```
which prints the same string, is ~20x faster.
```
$ time ./a.out > /dev/null
real 0m0.332s
user 0m0.328s
sys 0m0.000s
```
|
Performance
|
low
|
Major
|
240,614,711 |
react
|
Mouseenter event not triggered when cursor moves from disabled button
|
BUG.
Mouseenter event not triggered when cursor moves from disabled button
see [example](https://alfa-laboratory.github.io/arui-feather/styleguide/#playground/code=%3Cdiv%3E%0A%20%0A%20%20%20%20%3Cdiv%20className='row'%3E%0A%20%20%20%20%20%20%20%20Hover%20on%20%20right%20button%20and%20then%20move%20cursor%20to%20left%20button%0A%20%20%20%20%20%20%20%20%3Cdiv%3E%0A%20%20%20%20%20%20%20%20%20%20%20%20%3Cbutton%20onMouseEnter=%7B()=%3E%7Balert('Hey!');%7D%7D%20%3E%0A%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20Not%20Disabled%0A%20%20%20%20%20%20%20%20%20%20%20%20%3C/button%3E%0A%20%20%20%20%20%20%20%20%3C/div%3E%0A%20%20%20%20%20%20%20%20%3Cdiv%3E%0A%20%20%20%20%20%20%20%20%20%20%20%20%3Cbutton%20disabled=%7B%20true%20%7D%3EDisabled%3C/button%3E%0A%20%20%20%20%20%20%20%20%3C/div%3E%0A%20%20%20%20%3C/div%3E%0A%0A%20%20%20%20%3Cdiv%20className='row'%3E%0A%20%20%20%20%20%20%20%20Hover%20on%20%20right%20button%20and%20then%20move%20cursor%20to%20left%20button%0A%20%20%20%20%20%20%20%20%3Cdiv%3E%0A%20%20%20%20%20%20%20%20%20%20%20%20%3Cbutton%20onMouseEnter=%7B()=%3E%7Balert('Hey!');%7D%7D%20%3E%0A%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20Not%20Disabled%0A%20%20%20%20%20%20%20%20%20%20%20%20%3C/button%3E%0A%20%20%20%20%20%20%20%20%3C/div%3E%0A%20%20%20%20%20%20%20%20%3Cdiv%3E%0A%20%20%20%20%20%20%20%20%20%20%20%20%3Cbutton%3ENot%20Disabled%3C/button%3E%0A%20%20%20%20%20%20%20%20%3C/div%3E%0A%20%20%20%20%3C/div%3E%0A%20%0A%3C/div%3E)
**What is the expected behavior?**
Should trigger Mouseenter event
React 15.5.3
Latest Chrome
MacOs 10.12.5
|
Type: Bug,Component: DOM
|
medium
|
Critical
|
240,618,196 |
TypeScript
|
bang operator resets type inference
|
**TypeScript Version:** 2.3.4
**Code**
```ts
type Foo = {
foo:string;
};
function isFoo(arg?:any):arg is Foo|undefined{
return true;
}
function logic(arg?:object){
if (isFoo(arg)){
arg.foo; //Error:(..., 13) TS2532:Object is possibly 'undefined'.
arg!.foo; //Error:(..., 18) TS2339:Property 'foo' does not exist on type 'object'.
}
}
```
**Expected behavior:**
`arg!` should resolve to foo if it's already inferred that `arg is Foo|undefined`
**Actual behavior:**
`arg!` is resolved to `object`
|
Bug
|
low
|
Critical
|
240,641,301 |
You-Dont-Know-JS
|
Rewording might be needed - Async & Perf Book
|
Async & Perf book has:
This function is passed two function callbacks that act as...
I believe this needs rewording.
|
for second edition
|
low
|
Major
|
240,704,229 |
vscode
|
Show suggestion details always at the bottom
|
<!-- Do you have a question? Please ask it on http://stackoverflow.com/questions/tagged/vscode. -->
<!-- Use Help > Report Issues to prefill these. -->
- VSCode Version: 1.14.0 - Insiders (6afdf30 - Date 2017-07-05T08:33:33.146Z )
- OS Version: Windows 10
-- Sorry for the English, I used Google Translator --
As suggested by @ramya-rao-a after questioning this [comment](https://github.com/Microsoft/vscode/issues/18582#issuecomment-304643762)
Currently the same appears side, but I wish there was an option to put on the bottom.
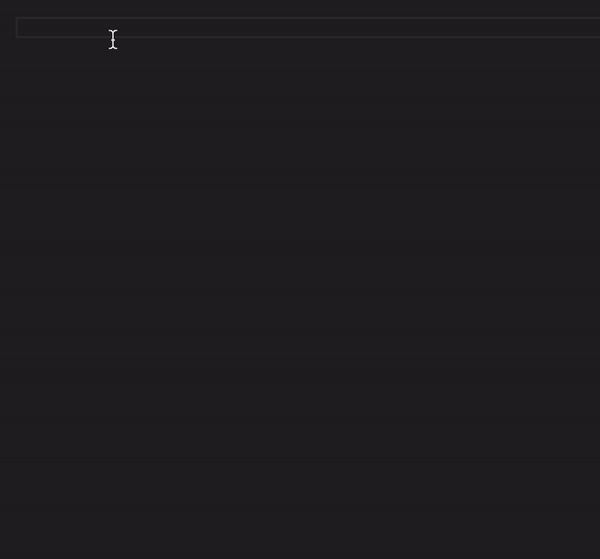
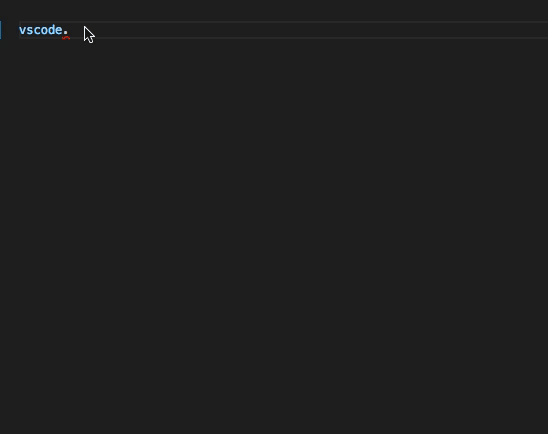
<!-- Launch with `code --disable-extensions` to check. -->
Reproduces without extensions: Yes
|
feature-request,suggest
|
low
|
Major
|
240,751,860 |
opencv
|
Bizzarre Bug in matchTemplate CV_TM_SQDIFF
|
#### System information (version)
- OpenCV => 3.2.0
- Operating System / Platform => Windows 64 Bit
- Compiler => Visual Studio 2013
#### Detailed description
Looking for multiple exact template match I'm trying CV_TM_SQDIFF. In case of exact match, score `0` is expected but result is not always the same between consecutive calls or matching position in the image.
#### Steps to reproduce
A bit hard to explain but following code can reproduce the error.
1. Use opencv-logo-small.png as template
1. Create a flat image BGR 640x480
1. Copy the template multiple times in the image at points Pi(x,y)
1. Perform CV_TM_SQDIFF
1. Repeat N times with different points set
The result in P(x,y) should be always 0 but not always it is.
```.cpp
int matchTemplateBug()
{
Mat T = imread("OpenCV/3.2.0/sources/opencv/doc/opencv-logo-small.png");
Rect R(Point(0, 0), T.size()); //a rect roi over the image
Mat I(480, 640, T.type());
// vector of exact match point
vector<vector < Point >> locationSets = {
{ Point(0, 0), Point(100, 30) },
{ Point(0, 0), Point(100, 30) },
{ Point(1, 1), Point(100, 30), Point(150, 200) },
{ Point(0, 0), Point(100, 30), Point(150, 200) },
{ Point(0, 0), Point(101, 31), Point(150, 200) },
};
//duplicate 1st set ->but get different result
locationSets.push_back(locationSets[0]);
//loop for all locations set
for (size_t n = 0; n < locationSets.size(); n++)
{
cout << endl << "Locations: ";
for (size_t i = 0; i < locationSets[n].size(); i++)
{
R = Rect(locationSets[n][i], T.size());
T.copyTo(I(R));
cout << locationSets[n][i] << "\t";
}
Mat result;
int match_method = CV_TM_SQDIFF;
matchTemplate(I, T, result, match_method);
cout << endl << " Scores: ";
for (size_t i = 0; i < locationSets[n].size(); i++)
{
cout << result(Rect(locationSets[n][i], Size(1, 1))) << "\t\t";
}
cout << endl;
}
return 0;
}
```
Below is the output (scores should be all 0)
Locations: [0, 0] [100, 30]
Scores: [0] [0]
Locations: [0, 0] [100, 30]
Scores: [0] [0]
Locations: [1, 1] [100, 30] [150, 200]
Scores: [12] [0] [0]
Locations: [0, 0] [100, 30] [150, 200]
Scores: [12] [0] [0]
Locations: [0, 0] [101, 31] [150, 200]
Scores: [0] [0] [0]
Locations: [0, 0] [100, 30]
Scores: [12] [0]
cc @snosov1 might this be related to #6919 (sorry @sovrasov for mistake cc)
|
bug,category: imgproc
|
low
|
Critical
|
240,834,026 |
kubernetes
|
service resource support set-based selector
|
<!-- This form is for bug reports and feature requests ONLY!
If you're looking for help check [Stack Overflow](https://stackoverflow.com/questions/tagged/kubernetes) and the [troubleshooting guide](https://kubernetes.io/docs/tasks/debug-application-cluster/troubleshooting/).
-->
**Is this a BUG REPORT or FEATURE REQUEST?**:
> /kind feature
**What you expected to happen**:
As the [doc][1] says:
> Newer resources, such as Job, Deployment, Replica Set, and Daemon Set, support set-based requirements as well.
[1]: https://kubernetes.io/docs/concepts/overview/working-with-objects/labels/
I want to get _endpoints_ by _service_ that select _pods_ with **key** = **v1** or **v2**. Is there any way to do so?
/sig api-machinery
|
sig/network,area/api,kind/feature,priority/important-longterm,triage/accepted
|
medium
|
Critical
|
240,838,414 |
opencv
|
GeneralHoughTransform does not work for trivial/Helloworld examples under samples/gpu/generalized_hough.cpp
|
<!--
If you have a question rather than reporting a bug please go to http://answers.opencv.org where you get much faster responses.
If you need further assistance please read [How To Contribute](https://github.com/opencv/opencv/wiki/How_to_contribute).
This is a template helping you to create an issue which can be processed as quickly as possible. This is the bug reporting section for the OpenCV library.
-->
##### System information (version)
- OpenCV => :3.2:
- Operating System / Platform => :Ubuntu 14.04:
- Compiler => :GNU 4.9.2:
##### Detailed description
Running the sample samples/gpu/generalized_hough.cpp for sample data under does not work. As you can see in attached images(out.png), its doing too many false detection.
##### Steps to reproduce
1. The sample is provided under samples/gpu/generalized_hough.cpp. The image and template are used as default if one does not provide them. I have tried with both --full option and without it.
The command is run as ./opencv_example --full --image=../data/pic1.png --template=../data/templ.png
2) The sample run finds the four matches, three of which are spurious.
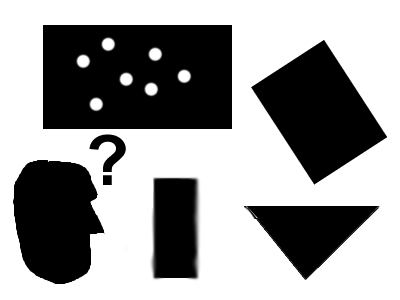
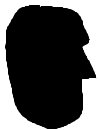
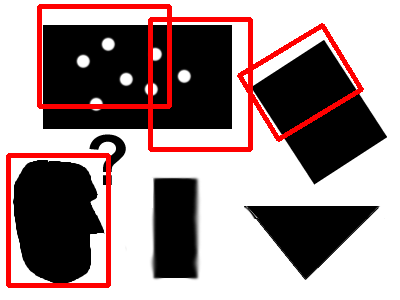
|
bug,category: imgproc
|
low
|
Critical
|
240,974,543 |
vscode
|
Change Vertical Scrollbar Width
|
Is there any way to change the width of the vertical scrollbars in Visual Studio Code, especially the one for the left panel?
I find it so thin that it's a bit fiddly to use.
|
feature-request,trackpad/scroll,unreleased
|
high
|
Critical
|
240,979,539 |
rust
|
Not all doc-comments on re-exports are displayed
|
In `nix` we're aliasing the `libc::termios` type using `pub use libc::termios as Termios`. That type has no documentation in `libc`, but we'd like to offer documentation for the type in our own crate. Specifying a doc comment above it doesn't support anything in the output. It'd be very useful if you could override documentation when aliasing types.
Originally posted at rust-lang/cargo#4250.
|
T-rustdoc,C-feature-request
|
low
|
Major
|
240,996,099 |
rust
|
incr. comp.: Access span information more carefully throughout the compiler.
|
Currently span information is accessed and copied rather haphazardly throughout the compiler which introduces lots of "false" dependency edges, because the span information is then never used or just used for error reporting. A few examples are:
- The code generating debuginfo for structs, enums, and unions fetches the `def_span` of the type in questions, just to throw it away again immediately.
- Queries collect spans from all over for possible cycle error reporting. In many cases these would not have to be access otherwise.
- MIR contains span information which is later threaded through to debuginfo, which forces us to have manual special casing for ICH computation depending on debuginfo being enabled or not.
- Type inference seems to pre-collect span information for error reporting. This information will not be used unless an actual error occurs.
I'm sure there are many other examples. Since spans are inherently unstable (adding a new-line somewhere will change all subsequent spans within the same file) this is a problem.
One possible solution would be to not store spans directly in the HIR and MIR (and other places) anymore but in a side-table that is part of the HIR map. Instead of a `Span` one would use and pass around a `SpanId` (which can just be a newtyped `HirId` or `NodeId`). Only when the `SpanId` is resolved to an actual `Span`, would we register a dependency on the thing the span originated from.
cc @nikomatsakis
|
C-cleanup,I-compiletime,T-compiler,A-incr-comp
|
low
|
Critical
|
241,015,848 |
TypeScript
|
narrowing in switch doesn't work with non-union types
|
```typescript
class A {
readonly kind = 'a';
}
type U = A; // <-- supposed to be a single case union
declare var u: U;
declare function never(never: never): never;
function fn() {
switch (u.kind) {
case 'a': return 1;
default: return never(u); // <-- u expected to be never, actually is A
}
}
```
|
Suggestion,In Discussion
|
medium
|
Major
|
241,017,199 |
TypeScript
|
narrowing in switch doesn't work with ambient enums
|
```typescript
declare global {
module g.m {
enum E { A, B }
}
}
declare var x: g.m.E;
declare function never(never: never): never;
function fn() {
switch (x) {
case g.m.E.A: return 1;
case g.m.E.B: return 2;
default: return never(x); // <-- x expected to be never, actual g.m.E
}
}
```
|
Bug
|
low
|
Minor
|
241,022,110 |
bitcoin
|
Think about tuning the script cache/sigcache ratios
|
After #10192, we have two separate caches, which are in memory blocks of equal size. This is likely not the best possible tuning, but sadly its tricky to get right because the sigcache is now used more as a DoS protection/reorg speedup and less as a cache used in normal operation. See-also, @JeremyRubin's suggestion of using the same table and further discussion at, eg, https://github.com/bitcoin/bitcoin/pull/10192#issuecomment-313334895.
|
Brainstorming,Resource usage
|
low
|
Minor
|
241,028,220 |
rust
|
Add `as_str` method for `str::Split(Whitespace)`
|
Consider the following use case:
```rust
// A command interpreter with a "print" command, which prints what comes after it
let mut words = line.split_whitespace();
match words.next() {
"print" => {
// How could we get the rest that comes after the command word?
// We could perhaps collect the words, and join them with a space, but that's lossy,
// and doesn't sound very efficient.
let rest;
println!("{}", rest),
}
unk => println!("Unknown command: {}", unk),
}
```
Some iterators, like `str::Chars` already have an `as_str` method. The only question is whether it is possible to implement `as_str` for the Split API, without making any breaking changes.
|
T-libs-api,C-feature-accepted
|
low
|
Major
|
241,111,369 |
go
|
cmd/compile: CSE some function calls args/results
|
```go
package p
var b bool
func f(x, y int) int {
var r int
if b {
r = g(x, y)
} else {
r = h(x, y)
}
return r
}
//go:noinline
func g(x, y int) int { return 0 }
//go:noinline
func h(x, y int) int { return 0 }
```
This kind of code ("call either g or h with the same args") is not uncommon. It is compiled suboptimally; there is lots of duplicate code to set up and read the stack:
```
"".f STEXT size=122 args=0x18 locals=0x20
0x0000 00000 (x.go:5) TEXT "".f(SB), $32-24
0x0000 00000 (x.go:5) MOVQ (TLS), CX
0x0009 00009 (x.go:5) CMPQ SP, 16(CX)
0x000d 00013 (x.go:5) JLS 115
0x000f 00015 (x.go:5) SUBQ $32, SP
0x0013 00019 (x.go:5) MOVQ BP, 24(SP)
0x0018 00024 (x.go:5) LEAQ 24(SP), BP
0x001d 00029 (x.go:5) FUNCDATA $0, gclocals·54241e171da8af6ae173d69da0236748(SB)
0x001d 00029 (x.go:5) FUNCDATA $1, gclocals·33cdeccccebe80329f1fdbee7f5874cb(SB)
0x001d 00029 (x.go:7) MOVBLZX "".b(SB), AX
0x0024 00036 (x.go:7) TESTB AL, AL
0x0026 00038 (x.go:7) JEQ 84
0x0028 00040 (x.go:7) MOVQ "".x+40(SP), AX
0x002d 00045 (x.go:8) MOVQ AX, (SP)
0x0031 00049 (x.go:8) MOVQ "".y+48(SP), AX
0x0036 00054 (x.go:8) MOVQ AX, 8(SP)
0x003b 00059 (x.go:8) PCDATA $0, $0
0x003b 00059 (x.go:8) CALL "".g(SB)
0x0040 00064 (x.go:8) MOVQ 16(SP), AX
0x0045 00069 (x.go:12) MOVQ AX, "".~r2+56(SP)
0x004a 00074 (x.go:12) MOVQ 24(SP), BP
0x004f 00079 (x.go:12) ADDQ $32, SP
0x0053 00083 (x.go:12) RET
0x0054 00084 (x.go:12) MOVQ "".x+40(SP), AX
0x0059 00089 (x.go:10) MOVQ AX, (SP)
0x005d 00093 (x.go:10) MOVQ "".y+48(SP), AX
0x0062 00098 (x.go:10) MOVQ AX, 8(SP)
0x0067 00103 (x.go:10) PCDATA $0, $0
0x0067 00103 (x.go:10) CALL "".h(SB)
0x006c 00108 (x.go:10) MOVQ 16(SP), AX
0x0071 00113 (x.go:7) JMP 69
0x0073 00115 (x.go:7) NOP
0x0073 00115 (x.go:5) PCDATA $0, $-1
0x0073 00115 (x.go:5) CALL runtime.morestack_noctxt(SB)
0x0078 00120 (x.go:5) JMP 0
```
Setting up the stack for the call and processing the return could be CSE'd to dominating and post-dominating blocks. In pseudo code:
```
func f(x, y int) int {
var r int
arg0 = x
arg1 = y
if b {
call g
} else {
call h
}
r = ret0
return r
}
```
I suspect that at least for function arguments, our current CSE pass would work, but for the prohibition on CSEing values of memory type.
At the end of the generic cse pass, the code above looks like:
```
f <T>
b1:
v1 = InitMem <mem>
v2 = SP <uintptr>
v3 = SB <uintptr>
v6 = Addr <*int> {~r2} v2
v7 = Arg <int> {x}
v8 = Arg <int> {y}
v10 = Addr <*bool> {"".b} v3
v11 = Load <bool> v10 v1
v13 = OffPtr <*int> [0] v2
v17 = OffPtr <*int> [8] v2
v20 = OffPtr <*int> [16] v2
If v11 -> b2 b4
b2: <- b1
v15 = Store <mem> {int} v13 v7 v1
v18 = Store <mem> {int} v17 v8 v15
v19 = StaticCall <mem> {"".g} [24] v18
v21 = Load <int> v20 v19
Plain -> b3
b3: <- b2 b4
v29 = Phi <int> v21 v28
v30 = Phi <mem> v19 v27
v31 = VarDef <mem> {~r2} v30
v32 = Store <mem> {int} v6 v29 v31
Ret v32
b4: <- b1
v24 = Store <mem> {int} v13 v7 v1
v26 = Store <mem> {int} v17 v8 v24
v27 = StaticCall <mem> {"".h} [24] v26
v28 = Load <int> v20 v27
Plain -> b3
```
Observe that v15/v24 and v18/v26 certainly look ripe for CSE.
Rolling back the prohibition on CSEing memory values would require care, though, since that helped a lot with CSE toolspeed problems. Continuing to prohibit CSE of calls might be a workable middle ground.
cc @randall77 @tzneal @dr2chase
|
Performance,ToolSpeed,compiler/runtime
|
low
|
Major
|
241,202,996 |
pytorch
|
Implement similar PyTorch function as model.summary() in keras?
|
`model.summary` in keras gives a very fine visualization of your model and it's very convenient when it comes to debugging the network. Can we try to implement something like it in PyTorch?
cc @ezyang @gchanan @zou3519 @bdhirsh @jbschlosser @albanD @mruberry
|
feature,module: nn,triaged,function request
|
high
|
Critical
|
241,209,270 |
flutter
|
`flutter run` does not terminate after failure to install
|
If I yank the cable from my device during installation (I know this isn't normal, but I'm simulating https://github.com/Dart-Code/Dart-Code/issues/329!) then flutter run sends an `app.stop` notification but does not terminate; leaving the process around.
Since this is not `daemon` and starts the app immediately at startup, I would expect that on app start it would terminate (and if I send a stop command, I believe it does).
```text
PS M:\Coding\Applications\flutter\examples\stocks> flutter run --machine
[{"event":"app.start","params":{"appId":"006f6f46-ba1e-4a68-aaf4-f3d53e9b8b80","deviceId":"ZY2224ZKJJ","directory":"M:\\Coding\\Applications\\flutter\\examples\\stocks","supportsRestart":true}}]
Launching lib/main.dart on XT1562 in debug mode...
[{"event":"app.progress","params":{"appId":"006f6f46-ba1e-4a68-aaf4-f3d53e9b8b80","id":"0","progressId":null,"message":"Initializing Gradle..."}}]
[{"event":"app.progress","params":{"appId":"006f6f46-ba1e-4a68-aaf4-f3d53e9b8b80","id":"0","progressId":null,"finished":true}}]
[{"event":"app.progress","params":{"appId":"006f6f46-ba1e-4a68-aaf4-f3d53e9b8b80","id":"1","progressId":null,"message":"Resolving dependencies..."}}]
// Cable yanked around here
[{"event":"app.progress","params":{"appId":"006f6f46-ba1e-4a68-aaf4-f3d53e9b8b80","id":"1","progressId":null,"finished":true}}]
[{"event":"app.progress","params":{"appId":"006f6f46-ba1e-4a68-aaf4-f3d53e9b8b80","id":"2","progressId":null,"message":"Running 'gradlew assembleDebug'..."}}]
[{"event":"app.progress","params":{"appId":"006f6f46-ba1e-4a68-aaf4-f3d53e9b8b80","id":"2","progressId":null,"finished":true}}]
Built build\app/outputs/apk/app-debug.apk (21.6MB).
// Error comes back with a stop notification
error: device 'ZY2224ZKJJ' not found
[{"event":"app.stop","params":{"appId":"006f6f46-ba1e-4a68-aaf4-f3d53e9b8b80","error":"Exit code 1 from: M:\\Apps\\Android\\platform-tools\\adb -s ZY2224ZKJJ shell pm list packages io.flutter.examples.stocks"}}]
// Process is still alive
```
Is my understanding correct - flutter should terminate here?
|
tool,P2,team-tool,triaged-tool
|
low
|
Critical
|
241,226,715 |
TypeScript
|
Array.isArray type narrows to any[] for ReadonlyArray<T>
|
<!-- BUGS: Please use this template. -->
<!-- QUESTIONS: This is not a general support forum! Ask Qs at http://stackoverflow.com/questions/tagged/typescript -->
<!-- SUGGESTIONS: See https://github.com/Microsoft/TypeScript-wiki/blob/master/Writing-Good-Design-Proposals.md -->
**TypeScript Version:** 2.4.1
**Code**
```ts
declare interface T {
foo: string;
}
const immutable: T | ReadonlyArray<T> = [];
if (Array.isArray(immutable)) {
const x = immutable; // Any[] - Should be ReadonlyArray<T>
}
const mutable: T | Array<T> = [];
if (Array.isArray(mutable)) {
const x = mutable; // T[]
}
```
**Expected behavior:** Should type narrow to ```ReadonlyArray<T>```, or at the very least ```T[]```.
**Actual behavior:** Narrows to ```any[]```. Doesn't trigger warnings in noImplicitAny mode either.
|
Suggestion,In Discussion,Domain: lib.d.ts,Fix Available
|
high
|
Critical
|
241,332,315 |
go
|
x/tools/present: add footer template
|
The Go Present tool lacks the ability to add a "footer" to each slide page. Having such functionality is required at some companies for internal presentations, e.g. for marking the slide deck as confidential. Such a template could also be used to expose other metadata about the specific slide such as the slide number.
I would like to implement a change to the slide format specification that enables the definition of a footer element.
It might also be useful to add the ability to turn off the footer for a specific slide.
I will update this proposal with specific proposed specification changes if this functionality is generally agreeable.
### Update - Proposal
I propose being able to optionally add to the Author block of the title slide:
```
<footer | [#]>
```
The author section may also contain a footer element. The foot can be broken into three segments with justifications using the pipe (`|`) character. The justifications are left, center, and right. Use `[#]` to denote the slide number.
Examples:
`<Go rules! | | [#]>`
```
Go rules! 1
```
`<|Go rules! | [#]>`
```
Go rules! 1
```
`<[#]>`
```
1
```
`<|[#]>`
```
1
```
I was unable to come up with a clean way to have some form of `nofooter` directive. I don't have strong feelings toward the chosen delimiters, they are merely what made sense to me.
|
Proposal,Proposal-Accepted,NeedsFix
|
low
|
Critical
|
241,373,339 |
opencv
|
documentation needs an extra step for running OpenCV from sbt
|
##### System information (version)
- OpenCV => 2.4
- Operating System / Platform => Linux 64 bit
- Compiler => sbt
##### Detailed description
In this document:
http://docs.opencv.org/2.4/doc/tutorials/introduction/desktop_java/java_dev_intro.html
Towards the end it says:
Note the call to System.loadLibrary(Core.NATIVE_LIBRARY_NAME). This command must be executed exactly once per Java process prior to using any native OpenCV methods. If you don’t call it, you will get UnsatisfiedLink errors. You will also get errors if you try to load OpenCV when it has already been loaded.
Adding just System.loadLibrary(Core.NATIVE_LIBRARY_NAME) is not enough.
Core.NATIVE_LIBRARY_NAME is just this:
opencv_java2413
Errors occur even if System.loadLibrary is added to code:
java.lang.UnsatisfiedLinkError: no opencv_java2413 in java.library.path
Full path to OpenCV system library folder (e.g. opencv/build/lib) is needed.
In ant project documentation there is this line:
ant -DocvJarDir=path/to/dir/containing/opencv-244.jar -DocvLibDir=path/to/dir/containing/opencv_java244/native/library
That's what's missing from sbt docs, how to pass full path to lib folder.
##### Steps to reproduce
Follow the document for sbt project build.
|
bug,category: documentation,affected: 2.4
|
low
|
Critical
|
241,400,999 |
TypeScript
|
this is any type in class property assignment to function
|
<!-- BUGS: Please use this template. -->
<!-- QUESTIONS: This is not a general support forum! Ask Qs at http://stackoverflow.com/questions/tagged/typescript -->
<!-- SUGGESTIONS: See https://github.com/Microsoft/TypeScript-wiki/blob/master/Writing-Good-Design-Proposals.md -->
From https://github.com/Microsoft/vscode/issues/30003
**TypeScript Version:** 2.4.1
**Code**
```ts
class AppComponent {
items = [ 'Angular 4', 'React', 'Underscore'];
foo = function () {
return this.items
}
}
```
**Expected behavior:**
The type of `this` in `foo` should be `AppComponent`
**Actual behavior:**
The type of `this` in `foo` is `any`
|
Suggestion,Awaiting More Feedback,VS Code Tracked,Domain: JavaScript
|
low
|
Critical
|
241,414,116 |
angular
|
Calling setValidators on a form control when control is nested as a component in form causes ExpressionChangedAfterItHasBeenCheckedError
|
<!--
PLEASE HELP US PROCESS GITHUB ISSUES FASTER BY PROVIDING THE FOLLOWING INFORMATION.
ISSUES MISSING IMPORTANT INFORMATION MIGHT BE CLOSED WITHOUT INVESTIGATION.
-->
## I'm submitting a ...
<!-- Check one of the following options with "x" -->
<pre><code>
[ ] Regression (behavior that used to work and stopped working in a new release)
[ X] Bug report <!-- Please search github for a similar issue or PR before submitting -->
[ ] Feature request
[ ] Documentation issue or request
[ ] Support request => Please do not submit support request here, instead see https://github.com/angular/angular/blob/master/CONTRIBUTING.md#question
</code></pre>
## Current behavior
<!-- Describe how the issue manifests. -->
Calling setValidators on a form control when control is nested as a component in form causes ExpressionChangedAfterItHasBeenCheckedError. Otherwise nesting formControls in components seems to work fine.
## Expected behavior
<!-- Describe what the desired behavior would be. -->
When calling myform.controls["my_control"].setValidators([Validators.required]); in a nested component of a reactive form I would expect no errors to occur, especially ExpressionChangedAfterItHasBeenCheckedError
## Minimal reproduction of the problem with instructions
<!--
For bug reports please provide the *STEPS TO REPRODUCE* and if possible a *MINIMAL DEMO* of the problem via
https://plnkr.co or similar (you can use this template as a starting point: http://plnkr.co/edit/tpl:AvJOMERrnz94ekVua0u5).
-->
## What is the motivation / use case for changing the behavior?
<!-- Describe the motivation or the concrete use case. -->
## Please tell us about your environment
<pre><code>
Angular version: ~4.2.0
<!-- Check whether this is still an issue in the most recent Angular version -->
Still an issue
Browser:
- [ X] Chrome (desktop) version XX
- [ ] Chrome (Android) version XX
- [ ] Chrome (iOS) version XX
- [ ] Firefox version XX
- [ ] Safari (desktop) version XX
- [ ] Safari (iOS) version XX
- [ ] IE version XX
- [ ] Edge version XX
For Tooling issues:
- Node version: 6.11.0 <!-- use `node --version` -->
- Platform: linux
Others:
<!-- Anything else relevant? Operating system version, IDE, package manager, HTTP server, ... -->
</code></pre>
|
type: bug/fix,freq3: high,area: forms,state: confirmed,design complexity: major,P4
|
medium
|
Critical
|
241,429,012 |
kubernetes
|
"Simulation" of scheduling behavior needs to work with modified scheduler policies, replacement schedulers, and multiple schedulers
|
This issue is forked off from #42002
#20204 moved the core scheduler predicates (the one used in kubelet admission) into a "library" function called GeneralPredicates so it could be shared with the default scheduler and with components that need to do "simulation" (need to know whether a particular pod will schedule) like rescheduler, cluster autoscaler, DaemonSet controller, etc.
This approach doesn't account for the fact that a cluster admin might replace the default scheduler with one whose predicates are stricter (or run alongside the default scheduler one whose predicates are stricter). It may give wrong results in such a situation. In fact this is even true if you use the default scheduler but use the scheduler policy config file to enable extra predicates (because such extra predicates will not show up in GeneralPredicates).
We should do two things
(1) to handle the "using default scheduler but enabled extra predicates" case, make the "predicate library" that the "simulators" use take the scheduler policy config into account
(2) to handle the "using a replacement scheduler with stricter predicates" case, either figure out how to make that work with the "predicate library" approach (but that seems impossible without requiring to recompile the components that do simulation, to link in the new predicate library), or require scheduler authors to have their schedulers (including default scheduler) export a "dry run" endpoint that takes a pod and tells you which nodes it can schedule onto (without modifying any of the scheduler's internal state). There is more discussion of this idea (suggested by @bgrant0607) in #42002. Note that it is a little more complicated than what I described because cluster autoscaler wants to know if a pending pod will schedule if a new node is hypothetically added to the cluster.
cc/ @kubernetes/sig-scheduling-feature-requests
cc/ @kubernetes/sig-autoscaling-feature-requests
cc/ @bgrant0607
|
sig/scheduling,sig/autoscaling,kind/feature,lifecycle/frozen
|
low
|
Major
|
241,444,174 |
rust
|
Unnecessary warning about panic at runtime
|
Given the following minimal code…
```rust
macro_rules! remainder {
($dividend:expr, $divisor:expr) => {
if $divisor == 0 {
0
} else {
$dividend % $divisor
}
};
}
fn main() {
#[allow(const_err)]
let _ = remainder!(12, 0);
}
```
… I get the following warning…
```
warning: this expression will panic at run-time
--> bad_macro.rs:6:13
|
6 | / $dividend % $divisor
7 | | }
8 | | };
9 | | }
... |
12 | | #[allow(const_err)]
13 | | let _ = remainder!(12, 0);
| | ---------------^-
| |_____________|______________|
| | attempt to calculate the remainder with a divisor of zero
| in this macro invocation
```
… although I obviously thought about it and protected myself against it.
Is there any possibility to deactivate this warning? If not, there should be.
I use the latest nightly (9b85e1cfa 2017-07-07).
|
C-enhancement,A-diagnostics,T-compiler,A-const-eval
|
low
|
Major
|
241,462,038 |
rust
|
Tracking issue for RFC 2033: Experimentally add coroutines to Rust
|
[RFC](https://github.com/rust-lang/rfcs/pull/2033).
This is an *experimental* RFC, which means that we have enough confidence in the overall direction that we're willing to land an early implementation to gain experience. **However, a complete RFC will be required before any stabilization**.
This issue tracks the initial implementation.
[**related issues**](https://github.com/rust-lang/rust/labels/A-coroutines)
|
T-lang,B-unstable,C-tracking-issue,A-coroutines,F-coroutines,S-tracking-design-concerns
|
high
|
Critical
|
241,464,083 |
TypeScript
|
Compiler hang when importing big JS file with --allowJs
|
**TypeScript Version:** 2.4.1
**Repro project**
When importing a big JS file (a working parser) (245 Kbytes commonjs format) (with `--allowJs` enabled) the compiler hangs forever. Minimal repro project provided: [tsc-hang-00](https://github.com/pjmolina/tsc-hang-00)
**Expected behavior:**
Compilations ends with or without errors.
**Actual behavior:**
Compilations hangs forever and keeps consuming CPU.
**Tests conditions**
- Windows 10, NodeJS 8.0, TS 2.4.1
- Travis: Linux Container, NodeJS 8.0, TS 2.4.1
|
Bug
|
low
|
Critical
|
241,486,167 |
neovim
|
Option to change behavior for pending (incomplete) mappings
|
It's a feature request:
Run this: `:cmap vv <esc>` and `:set tm=10000` now regardless of `showcmd` status if you press `v` in cmdline it shows the entered `v` (overwriting the character behind it) for 10 sec. It'd be great if cmdline respected `showcmd` state or there were another option to disable displaying pressed keys in operator pending mode in cmdline.
|
enhancement
|
low
|
Major
|
241,533,297 |
go
|
net/http: read: connection reset by peer under high load
|
### What version of Go are you using (`go version`)?
go1.8.3
### What operating system and processor architecture are you using (`go env`)?
GOARCH="amd64"
GOOS="linux"
### What did you do?
1. Edit [/etc/sysctl.conf](https://gist.github.com/liranp/50c90a441f5c29b41b63cdacf745e949#file-sysctl-conf) and reload with `sysctl -p`.
2. Edit [/etc/security/limits.conf](https://gist.github.com/liranp/50c90a441f5c29b41b63cdacf745e949#file-limits-conf).
3. Execute the following server and client (on different hosts) to simulate multiple HTTP requests:
Server https://play.golang.org/p/B3UCQ_4mWm
Client https://play.golang.org/p/_XQRqp8K5b
### What did you expect to see?
No errors at all.
### What did you see instead?
Errors like the following:
```bash
Get http://<addr>:8080: read tcp [::1]:65244->[::1]:8080: read: connection reset by peer
Get http://<addr>:8080: write tcp [::1]:62575->[::1]:8080: write: broken pipe
Get http://<addr>:8080: dial tcp [::1]:8080: getsockopt: connection reset by peer
Get http://<addr>:8080: readLoopPeekFailLocked: read tcp [::1]:51466->[::1]:8080: read: connection reset by peer
```
|
help wanted,NeedsInvestigation
|
high
|
Critical
|
241,536,797 |
youtube-dl
|
Updating in Windows using -U or --update switch results in cmd crash
|
Updating in windows causes cmd to crash and I believe the problem is in the batch file code.
`
@echo off
echo Waiting for file handle to be closed ...
ping 127.0.0.1 -n 5 -w 1000 > NUL
move /Y "C:\Michael Hall\Workspace\Development\Old Projects\MediaDownloader\MediaDownloader\bin\Release\youtube-dl.exe.new" "C:\Michael Hall\Workspace\Development\Old Projects\MediaDownloader\MediaDownloader\bin\Release\youtube-dl.exe" > NUL
echo Updated youtube-dl to version 2017.07.09.
start /b "" cmd /c del "%~f0"&exit /b"
`
Should not the last line have 2 & and spaces? Eg. start /b "" cmd /c del "%~f0" && exit /b" or even better:
start /b "" cmd /c del "%~f0"
exit
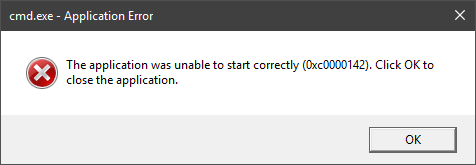
Honestly I don't know but it gets stuck in cmd window and throws an error if using C# for example for standard output;
|
cant-reproduce
|
low
|
Critical
|
241,588,730 |
angular
|
Access DOM element of FormControl programatically
|
I have searched Google, Angular.io docs, and the source code and I could not find if this is currently possible in the latest version of Angular.
I would like to propose the ability to access the DOM element that a FormControl is bound to when using the formControlName directive. It would be publicly readonly. If a FormControl is not bound using the formControlName directive, then the property would be null.
The rationale behind this request is so that the state of the element can be managed from the class, without having to expose any of the view's markup (i.e. the id attribute or any other xpath selector).
An example use case would be a situation where you want to listen for the onBlur/onFocus events so that, for example, when a user selects a control, some JavaScript can perform an animation/highlight to show the user the field currently being edited – conversely, the onBlur can undo those changes except for if the form's value exists and/or was modified.
For me, I would like to take advantage of RxJS's Observable on those events, without having to litter my HTML markup for each event I want to listen to for each of my elements (medium-sized form). When focusing on an element I want to be able to perform an animation to move the label (which starts at the same visual height as the form control) above the form control so the user can type. The form control would also have a bottom border that would change color to show it's the selected input. When the field is blurred, if there is no value, I want to move the label back to its initial locate and remove the bottom border.
Where this begins to become complex are when certain controls need extra behavior – a date picker that "opens" automatically when the field is focused, and closes automatically when focus is lost. A select drop down that, based on selection, may need to affect (show/hide) other form controls.
Thank you for your time. Please let me know if I need to clarify any of the above.
|
feature,state: Needs Design,freq2: medium,area: forms,feature: under consideration
|
high
|
Critical
|
241,590,232 |
go
|
cmd/compile: late nilcheck elim doesn't elim later nilcheck
|
Reproduce:
1. Disable the regular (early) nilcheckelim pass.
2. `go tool compile -d=nil x.go` this code:
```go
package p
//go:noinline
func fx10k() *[10000]int { return nil }
func f3(x *[10000]int) {
x = fx10k()
_ = x[9999]
_ = x[9999]
}
```
Result:
```
x.go:8:7: removed nil check
x.go:9:7: generated nil check
```
This is backwards. We should generate the first nil check and remove the second one, on pain of panicking on the wrong line.
I'm not sure whether this impacts any code in practice right now. Obvious reproducers like the one above already have their duplicate nil checks removed by the early nilcheck pass. I noticed it when implementing the TODO at the end of nilcheck2 caused the wrong nil checks to be eliminated from test/nilptr3.go.
Opinions about the correct fix are welcome.
cc @randall77
|
compiler/runtime
|
low
|
Minor
|
241,604,344 |
vscode
|
Cannot move last cursor position when using multi-cursor
|
Hi,
- VSCode Version: 1.13.1
- OS Version: macOS Sierra 10.12.5
I went through the options available in settings and keyboard shortcuts but didn't find an option to enable this. I also posted on SO tagging `vscode` but no one seems to have an answer for this question.
Is there a way to enable this option?
|
feature-request,editor-multicursor
|
low
|
Minor
|
241,657,798 |
youtube-dl
|
[acloud.guru] Site support request
|
I am not able to download cloud.guru videos. It is showing the error "Unsupported URL"
|
site-support-request,account-needed
|
low
|
Critical
|
241,670,907 |
flutter
|
TabBarView should give priority to vertical scrolling over horizontal
|
I faced a problem when using TabBarView that includes multiple ScrollViews.
I try to scroll vertically, but sometimes fail. It is because the thumb moves diagonally when operating with one hand.
I want to prioritize vertical (ie inner) scroll than horizontal. Because in mobile apps, vertical scrolling is more frequently operation than horizontal.
TabBarView uses HorizontalDragGestureRecognizer internally. It judges whether the delta of horizontal movement is sufficient.
https://github.com/flutter/flutter/blob/f64bfba860d143f3f3b9d8970a15c088b0d9599d/packages/flutter/lib/src/gestures/monodrag.dart#L259
The delta is same value in both VerticalDragGestureRecognizer and HorizontalDragGestureRecognizer. I think that changing that value will work for TabBarView/PageView.
In addition, I found `kPagingTouchSlop`.
https://github.com/flutter/flutter/blob/438f4df00c64c304d037b7cf3d6678199816ab18/packages/flutter/lib/src/gestures/constants.dart#L62
```dart
/// The distance a touch has to travel for us to be confident that the gesture
/// is a paging gesture. (Currently not used, because paging uses a regular drag
/// gesture, which uses kTouchSlop.)
// TODO(ianh): Create variants of HorizontalDragGestureRecognizer et al for
// paging, which use this constant.
const double kPagingTouchSlop = kTouchSlop * 2.0; // Logical pixels
```
## Flutter Doctor
```
[✓] Flutter (on Mac OS X 10.12.5 16F73, locale ja-JP, channel master)
• Flutter at /Applications/flutter
• Framework revision 4891506931 (2 days ago), 2017-07-07 16:00:45 -0700
• Engine revision 8ad1576f27
• Tools Dart version 1.25.0-dev.4.0
```
|
c: new feature,framework,f: scrolling,f: gestures,customer: crowd,P3,team-framework,triaged-framework
|
low
|
Major
|
241,794,268 |
TypeScript
|
Feature: Composing spread operator on Union types and static_key function to enable generic capability
|
Related issues: #13542 #15759 (slightly different take and example on closed issues)
I originally posted on issue #15759 but decided that they were different enough to be looked at separately and that issue doesn't address a generic capability. Look back at https://github.com/Microsoft/TypeScript/issues/15759#issuecomment-314157616 for clunky workaround examples.
**Proposal**
1. Add spread operator `...` to string literal union types acting as an string array or generator. Type `T` applying spread operator will result in type `T[]`. For example:
```
type Group = 'red' | 'blue' | 'green';
const groups = ...Group; // groups has type of Group[] with values ['red', 'green', 'blue']
```
2. Add static function `static_key` or `keyof` that transforms a static interface to a string literal union of all combined types. For example:
```
interface Groups<T, U, V> {
red: T;
green: U;
blue: V;
}
type groups = static_key(Groups); // 'red' | 'blue' | 'green'
```
3. Composing both can result in powerful generic operations. I feel that this could bring a unique capability that string enums could not and may have been overlooked. It would be very powerful for a `Record` type or interface where all fields are the same type. Here is an example:
```
interface GroupDescription {
name: string;
description: string;
}
type Group = 'red' | 'blue' | 'green';
type Groups = { [K in Group]: GroupDescription };
function initGroups(groups: Groups) {
for(const group of ...static_key(Groups)) { // group would be type Group or 'red' | 'blue' | 'green'
processGroup(name); //Generically Process property
}
}
function processGroup(group: Group) { }
```
I noticed I don't need `...static_key` but instead directly use the spread operator on the union type `...Group`. The point is that they compose well together and they add generic capability.
|
Suggestion,Needs Proposal
|
medium
|
Critical
|
241,797,629 |
go
|
sync: add examples for Map
|
It would be nice if there were usage examples for sync.Map, but there are not currently any.
- Can keys be ordinary values like strings, or should they be custom types, a la context.WithValue?
- Should I always store a pointer to a value, or can I store a value like a string? If the former how should I do it?
|
Documentation,help wanted,NeedsFix,compiler/runtime
|
low
|
Major
|
241,805,251 |
create-react-app
|
Enabling 2-way PKI authentication
|
I need 2-way authentication to be configurable because my company requires all internal webapps to use it.
The proposal is three-fold. I want the following to be configurable:
* Configure the webapp to request the client's certificate,
* Once the webapp receives the certificate pass information about the cert to the expressjs server,
* Make the webapps' certificate and key configurable.
Background on this:
* Two-way authentication involves the browser/client sending a user's certificate to the back-end.
* Passport, for example, has a strategy for this: https://github.com/ripjar/passport-client-cert
* In proxying environments, it's acceptable for the webapp/frontend to send certificate attributes to the backend
* This was discussed here, #1413, which provides some useful links:
* http://www.zeitoun.net/articles/client-certificate-x509-authentication-behind-reverse-proxy/start
* https://serverfault.com/questions/622855/nginx-proxy-to-back-end-with-ssl-client-certificate-authentication
* https://lists.gt.net/apache/users/350827
Without the above I can't easily test my passport setup and can't easily test my custom authentication code until I build for production.
My open questions are:
* Is there any way to customize the webapp's configuration? It appears to me we have to rely on create-react-app to do it for us.
* My understanding from #1413 is that WDS will also need modifications in order for the webapp to pass cert attributes to the backend. I'll need to raise an issue for that over at WDS. Is this correct?
|
issue: proposal
|
medium
|
Major
|
241,902,734 |
electron
|
Feature request: Access to "user gesture" flag, especially in 'new-window' event
|
Knowing whether the user initiated the `new-window` event is important for blocking unwanted popups in a webview. Without it, an untrusted page can call `window.open()` or `anchorTag.click()` to trigger popups at will.
In `webContents.executeJavascript`, we have a `userGesture` parameter which overrides the flag indicating whether the user triggered an event.
Is it possible for the application to get access to the state of that flag via a function call - something like `webContents.hasUserGesture()`? If not, could we get access to it in the `new-window` event via an attribute, `event.hasUserGesture` ?
(I can make a PR if somebody can assure me this is doable, and point to relevant APIs.)
|
enhancement :sparkles:
|
low
|
Minor
|
241,925,479 |
rust
|
`cannot move out of borrowed content` error is bad when self is borrowed
|
E.g., consider a struct with a field `build_queue` which is owned. In a `&self` function I call `request_build` which takes `self` by value. I get the error:
```
error[E0507]: cannot move out of borrowed content
--> src/actions/mod.rs:151:9
|
151 | self.build_queue.request_build(project_path, priority, move |result| {
| ^^^^ cannot move out of borrowed content
```
This is not very useful since it does not indicate why a move is happening and the squiggle is indicating the wrong thing. I think the squiggle should be under `self.build_queue` rather than just `self` and that there should be a note referring to the definition of `request_build` and indicating the by-value `self` which causes the move.
<!-- TRIAGEBOT_START -->
<!-- TRIAGEBOT_ASSIGN_START -->
<!-- TRIAGEBOT_ASSIGN_DATA_START$${"user":"ibraheemdev"}$$TRIAGEBOT_ASSIGN_DATA_END -->
<!-- TRIAGEBOT_ASSIGN_END -->
<!-- TRIAGEBOT_END -->
|
C-enhancement,A-diagnostics,A-borrow-checker,T-compiler
|
low
|
Critical
|
241,943,088 |
go
|
x/net/http2: connecting to non-compliant HTTP2 server returns Client.Timeout exceeded; fallback to HTTP/1?
|
### What version of Go are you using (`go version`)?
```
go version go1.8.3 darwin/amd64
```
### What operating system and processor architecture are you using (`go env`)?
```
GOARCH="amd64"
GOBIN=""
GOEXE=""
GOHOSTARCH="amd64"
GOHOSTOS="darwin"
GOOS="darwin"
GOPATH="/Users/tkng"
GORACE=""
GOROOT="/usr/local/Cellar/go/1.8.3/libexec"
GOTOOLDIR="/usr/local/Cellar/go/1.8.3/libexec/pkg/tool/darwin_amd64"
GCCGO="gccgo"
CC="clang"
GOGCCFLAGS="-fPIC -m64 -pthread -fno-caret-diagnostics -Qunused-arguments -fmessage-length=0 -fdebug-prefix-map=/var/folders/z1/nc5xfnrs71g2zxr_chmshx3h0000gp/T/go-build993898931=/tmp/go-build -gno-record-gcc-switches -fno-common"
CXX="clang++"
CGO_ENABLED="1"
PKG_CONFIG="pkg-config"
CGO_CFLAGS="-g -O2"
CGO_CPPFLAGS=""
CGO_CXXFLAGS="-g -O2"
CGO_FFLAGS="-g -O2"
CGO_LDFLAGS="-g -O2"
```
### What did you do?
Following snippet code should be enough to reproduce the problem.
https://play.golang.org/p/PmZWp6NJlq
If I disabled HTTP2 by the environment variable `GODEBUG=http2client=0`, then I see no error. Hence I guess this issue is related to HTTP2. This is might be the same as #13959, but I'm not sure.
Also, I'm not sure this is the problem of golang's HTTP2 client library, or the problem of server side. (In most case, HTTP2 client works nicely.)
### What did you expect to see?
I can connect to the server (by http2, or falling back to http1.1). At least, I want to see a more suggestive error message.
### What did you see instead?
I saw an error message like following:
```
2017/07/11 15:22:01 http.Get failed, error: Get https://precious.jp: net/http: request canceled (Client.Timeout exceeded while awaiting headers)
```
|
NeedsFix
|
medium
|
Critical
|
241,961,611 |
rust
|
Add syntax highlighting for TOML to rustdoc
|
Since TOML is such an essential part of the Rust ecosystem, it’s probably worth adding syntax highlighting for this file format. [Here](https://docs.rs/futures/0.1.14/futures/#installation) is an example of a code block that is currently ignored, syntax-highlighting-wise, when generating the documentation.
|
T-rustdoc,C-feature-request
|
medium
|
Major
|
241,966,036 |
rust
|
Improve error message on namespace collision
|
```rust
struct Model;
fn model(model: Model) -> Model {
model
}
fn main() {
let mut model = Model;
model = model(model);
}
```
The error message is:
```
error: expected function, found `Model`
--> <anon>:9:13
|
9 | model = model(model);
| ^^^^^^^^^^^^
|
note: defined here
--> <anon>:8:9
|
8 | let mut model = Model;
| ^^^^^^^^^
error: aborting due to previous error
```
I guess, the compiler should suggest you to avoid using the same names for functions and variables.
|
C-enhancement,A-diagnostics,T-compiler,D-papercut
|
low
|
Critical
|
242,017,630 |
TypeScript
|
Mixed es5/es2015 code with mixin classes causes runtime errors
|
**TypeScript Version:** 2.4.1 (but likely anything since 2.2)
When utilising code targeted at ES5 mixed with code targeted at ES6 causes a [runtime error when using mixin classes](https://jsfiddle.net/kitsonk/pn2f3ad0/). The real world use case was using a library that was targeted at ES5 for distribution compatibility reasons while downstream code is being targeted at ES2017 because of running in a known limited environment.
**Code**
*Tagged.ts*
```ts
// @target: es5
export interface Constructor<T> {
new(...args: any[]): T;
prototype: T;
}
export default function Tagged<T extends Constructor<{}>>(Base: T) {
return class extends Base {
_tag = '';
}
}
```
*TaggedExample.ts*
```ts
// @target: es2015
import Tagged from './Tagged';
class Example {
log() {
console.log('Hello World!');
}
}
const TaggedExample = Tagged(Example);
const taggedExample = new TaggedExample(); // Uncaught TypeError: Class constructor Example cannot be invoked without 'new'
```
**Expected behavior:**
No run-time errors.
**Actual behavior:**
A runtime error of `Uncaught TypeError: Class constructor Example cannot be invoked without 'new'`.
|
Suggestion,Help Wanted,Effort: Moderate,Awaiting More Feedback
|
medium
|
Critical
|
242,029,054 |
react
|
Remove unstable_renderIntoContainer
|
My hunch is we want to remove it before 16 because `unstable_createPortal` accomplishes the same thing. I remember `unstable_renderIntoContainer` adding a bunch of complexity that would be nice to get rid of before committing to support it for another release cycle.
|
Type: Discussion,Component: Reconciler,Type: Breaking Change,React Core Team
|
medium
|
Major
|
242,114,810 |
flutter
|
Document the extended layer cake
|
See https://github.com/flutter/flutter/pull/11135#discussion_r126579601
|
team,framework,a: accessibility,d: api docs,P3,team-framework,triaged-framework
|
low
|
Minor
|
242,119,735 |
go
|
cmd/link: support msvc object files
|
I understand that the go linker cannot currently link msvc object files and also recognize that this issue is likely to be a low priority. However, it would be nice to support this because it would somewhat simplify windows workflow. This issue is mainly to understand how much effort this would be and/or what would be required.
|
OS-Windows,Builders,NeedsInvestigation,FeatureRequest,compiler/runtime
|
high
|
Critical
|
242,138,490 |
vscode
|
Autoindent inserts extra undo item, requires double undo after paste
|
- VSCode Version: 1.14
- OS Version: macOS 10.12.5
Here's an example of pasting something, then hitting cmd-Z to undo. As you can see, it first undoes one indentation, then undoes the paste. That's not right, since my paste was a single command, so a single undo should undo it.
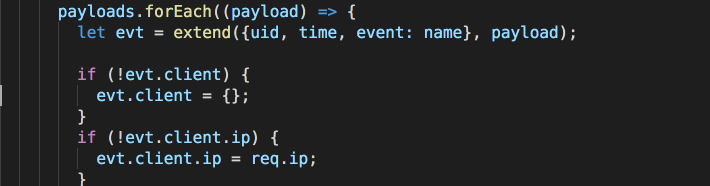
Steps to Reproduce:
1. Copy something.
2. Go into a block that has a deeper indentation level.
3. Hit cmd-V.
4. Hit cmd-Z.
5. The paste remains, but one indent level is subtracted.
Reproduces without extensions: Yes
|
editor-autoindent,under-discussion,undo-redo
|
medium
|
Major
|
242,176,274 |
rust
|
Unclear error note due to use of underscores when unifying paremeters to a single generic type
|
[Playground link](https://is.gd/sMns3l)
When I compile the following code:
```
fn test<T>(x: (T, Vec<T>)) {}
fn main() {
let param = ("hi", vec!(1, 2));
test(param);
}
```
I get the following error message:
```
rustc 1.18.0 (03fc9d622 2017-06-06)
error[E0308]: mismatched types
--> <anon>:5:10
|
5 | test(param);
| ^^^^^ expected &str, found integral variable
|
= note: expected type `(_, std::vec::Vec<_>)`
found type `(&str, std::vec::Vec<{integer}>)`
```
It is unclear that the types in the note aren't matching. `(_, std::vec::Vec<_>)` seems like it should correspond to `(T, std::vec::Vec<S>)`, which would unify with `(&str, std::vec::Vec<{integer}>)` just fine. The overall error message does not provide the desired context to fix the problem, and requires that you look up what the specific types represented by the underscores are to understand why it is failing to typecheck.
|
C-enhancement,A-diagnostics,T-compiler,D-papercut
|
low
|
Critical
|
242,226,656 |
TypeScript
|
JavaScript heap out of memory for 10s of MB of source
|
<!-- BUGS: Please use this template. -->
<!-- QUESTIONS: This is not a general support forum! Ask Qs at http://stackoverflow.com/questions/tagged/typescript -->
<!-- SUGGESTIONS: See https://github.com/Microsoft/TypeScript-wiki/blob/master/Writing-Good-Design-Proposals.md -->
**TypeScript Version:** 2.4.1 / nightly (2.5.0-dev.20170707)
**Code**
gulpfile.js
```js
// A *self-contained* demonstration of the problem follows...
gulp.task('compile-ts', () => {
const ts = require('gulp-typescript');
const tsProject = ts.createProject('./tsconfig.json');
tsProject.options.module = 1;
const dest = tsProject.options.outDir;
return gulp.src('./src/**/*.ts')
.pipe(tsProject())
.pipe(gulp.dest(dest));
});
```
tsconfig.json
```json
{
"include": [
"src"
],
"compilerOptions": {
"module": "commonjs",
"target": "esnext",
"noImplicitAny": false,
"sourceMap": false,
"emitDecoratorMetadata": true,
"experimentalDecorators": true,
"strictNullChecks": false,
"noImplicitThis": true,
"rootDir": "./src/",
"rootDirs": [],
"allowJs": false,
"allowUnreachableCode": false,
"allowUnusedLabels": false,
"alwaysStrict": true,
"baseUrl": "",
"charset": "utf8",
"declaration": false,
"inlineSourceMap": false,
"allowSyntheticDefaultImports": false,
"diagnostics": false,
"emitBOM": false,
"forceConsistentCasingInFileNames": false,
"importHelpers": false,
"inlineSources": false,
"isolatedModules": false,
"lib": [
"es6",
"es7",
"dom"
],
"listFiles": true, // default false
"listEmittedFiles": true, // default false
"locale": "zh_CN",
"newLine": "CRLF",
"noEmit": false,
"moduleResolution": "node",
"noEmitHelpers": false,
"noEmitOnError": false,
"noImplicitReturns": false,
"noImplicitUseStrict": false,
"maxNodeModuleJsDepth": 0,
"noLib": false,
"outDir": "./dist/",
"noFallthroughCasesInSwitch": false,
"noResolve": false,
"noUnusedLocals": false,
"noUnusedParameters": false,
"paths": {},
"preserveConstEnums": false,
"pretty": true,
"removeComments": false,
"skipDefaultLibCheck": true, // default false
"skipLibCheck": true, // default false
"stripInternal": false,
"suppressExcessPropertyErrors": false,
"suppressImplicitAnyIndexErrors": true, // default false
"traceResolution": true, // default false
"typeRoots": [],
"types": [],
"watch": false
}
}
```
**Expected behavior:**
**Actual behavior:**
Exception i got:
```bash
<--- Last few GCs --->
[24442:0x2def9d0] 58440 ms: Mark-sweep 1400.1 (1465.9) -> 1400.1 (1465.9) MB, 1948.3 / 0.0 ms allocation failure GC in old space requested
[24442:0x2def9d0] 60312 ms: Mark-sweep 1400.1 (1465.9) -> 1400.1 (1434.9) MB, 1871.6 / 0.0 ms last resort
[24442:0x2def9d0] 62184 ms: Mark-sweep 1400.1 (1434.9) -> 1400.0 (1434.9) MB, 1871.8 / 0.0 ms last resort
<--- JS stacktrace --->
==== JS stack trace =========================================
Security context: 0x3d1f48829891 <JS Object>
2: write [/home/taoqf/feidao/temp/boai-h5-6/1/node_modules/typescript/lib/typescript.js:~9035] [pc=0x3cfdedb6fe70](this=0x1857e443e689 <an Object with map 0xddd4399bc9>,s=0x3c6749682c29 <String[4]: "id">)
3: pipelineEmitExpression [/home/taoqf/feidao/temp/boai-h5-6/1/node_modules/typescript/lib/typescript.js:~66807] [pc=0x3cfdedbd085f](this=0xf51a038dbf9 <JS Global Object>,node=0x29110b...
FATAL ERROR: CALL_AND_RETRY_LAST Allocation failed - JavaScript heap out of memory
1: node::Abort() [gulp]
2: 0x13647ec [gulp]
3: v8::Utils::ReportOOMFailure(char const*, bool) [gulp]
4: v8::internal::V8::FatalProcessOutOfMemory(char const*, bool) [gulp]
5: v8::internal::Factory::NewFillerObject(int, bool, v8::internal::AllocationSpace) [gulp]
6: v8::internal::Runtime_AllocateInTargetSpace(int, v8::internal::Object**, v8::internal::Isolate*) [gulp]
7: 0x3cfded28437d
Aborted
```
**More**
- `tsc` works fine.
- do not depend on `fp-ts`, `fantasyland` and `monet`
- if i compile my ts files separately(1 ts file per time), also there is no exception.
- i have searched all the issues already and found no clue. like https://github.com/Microsoft/TypeScript/issues/14628#issuecomment-314133082
|
Bug
|
medium
|
Critical
|
242,512,086 |
TypeScript
|
Add capability of transforming and emitting JSDoc comments
|
So I am trying to create a new documentation generator for TypeScript together with @BurtHarris. Currently it does not do much; I've been mainly tinkering a bit with the compiler API to see what it is capable of. You will be able to find it [here](https://github.com/TypeForce/tsdoc) when it's finished.
One of the things we thought about was the ability to 'target' JSDoc comments using the type information provided by TypeScript. I've been investigating how to tackle the issue. Ideally, it would use the transformers the compiler API has to offer but of all the functions provided in `factory.ts`, the ones that came closest were `addSyntheticLeadingComment`, `setSyntheticLeadingComments`, `setSyntheticTrailingComments` and `addSyntheticTrailingComment`; none of which provide the means to create complete synthetic JSDoc tags and comments.
So with this issue I'm actually asking the status-quo of this feature. Is it still possible to get this on the [roadmap](https://github.com/Microsoft/TypeScript/wiki/Roadmap)? If so, can I help? If not, is there a possibility of a patch that makes it possible to implement this as a third-party module?
### Related issues
- [Generate doc comments in emitted JS](https://github.com/Microsoft/TypeScript/issues/10)
- [Cannot add or modify JSDoc with custom transformers](https://github.com/Microsoft/TypeScript/issues/15639)
- [Expose public API for transformation](https://github.com/Microsoft/TypeScript/pull/13940)
- [Replace emitter with syntax tree transformations and a simplified node emitter](https://github.com/Microsoft/TypeScript/issues/5595)
### Previous attempts
- [spatools/ts2jsdoc](https://github.com/spatools/ts2jsdoc)
- [develar/ts2jsdoc](https://github.com/develar/ts2jsdoc)
|
Suggestion,In Discussion,API
|
medium
|
Major
|
242,522,849 |
TypeScript
|
improve docs of compiler APIs
|
hi,
i'm trying to implement an instrumenter on top of typescript compiler APIs.
i cannot find a "real" documentation beside of this [wiki page](https://github.com/Microsoft/TypeScript/wiki/Using-the-Compiler-API) but i think that it should be upgraded from a single wiki page to a dedicated section with examples for:
- creating new nodes (e.g. create a brand new statement and attach it to an existing function body)
- updating existing nodes (e.g. patching a statement)
- add types declarations (e.g. add arbitrary types to a function declaration)
- _todo..._
what do you think about this?
EDIT: i've found this [nice article](http://blog.scottlogic.com/2017/05/02/typescript-compiler-api-revisited.html) that shows some really useful code.
EDIT: i've found this [nice project](https://github.com/dsherret/ts-simple-ast) that can help to understand how to use the compiler apis for more advanced use cases.
|
Docs
|
low
|
Major
|
242,537,246 |
TypeScript
|
Add plugins property on TS Server open request
|
## Problem
VSCode allows TSServer plugin extensions to register themselves for language modes besides javascript and typescript. The angular extension for example can register the angular TSServer plugin for the `ng-html` language. When an `ng-html` file is sent to TS and the angular plugin is not active, Typescript will treat the file contents as typescript code, resulting in a number of syntax errors
## Proposal
Add an optional `plugins` property on TSServer `open` requests.
```ts
interface OpenRequestArgs {
...,
plugins?: string[]
}
```
`plugins` would be a list of tsserver plugin package identifiers.
On the TypeScript side, the new logic for handling the `plugins` property on `open` requests would look something like:
* If `plugins` is undefined, the `open` request behaves the same as it does currently.
* If `plugins` is an array, TypeScript checks to see if any of the plugins it has loaded are in the `plugins`.
* When no match is found, the opened file is ignored by the TSServer
* If a match is found, the opened file is handled by TypeScript. The plugin would be responsible for disabling the standard TS features in the file
## Open Questions
**What does ignoring a file mean?**
What happens when `plugins` is provided but no match is found against the loaded plugins on the TSServer? Two options I see:
1. TS keeps the file open and VSCode continues sending update events about it, but TS never actually generate any events or fufills any requests against it.
2. TS tells VSCode that it doesn't care about this file, perhaps by returning an error from `open` VSCode would no longer send sync events about the file or send TS requests about it
**scriptKindName**
`OpenRequestArgs` includes an optional field `scriptKindName?: ScriptKindName`.
```ts
type ScriptKindName = "TS" | "JS" | "TSX" | "JSX";
```
Should a new kind for external files be added?
```ts
type ScriptKindName = "TS" | "JS" | "TSX" | "JSX" | "EXTERNAL";
```
|
Bug,VS Code Tracked,Domain: TSServer
|
medium
|
Critical
|
242,563,967 |
opencv
|
Unable to use detected keypoints by FastFeatureDetector to compute with ORB (CUDA version)
|
##### System information (version)
- OpenCV => Latest
- Operating System / Platform => Ubuntu 16.04
- Compiler => GCC 5.4
##### Detailed description
I am unable to use the detected keypoints found by FastFeatureDetector to compute the descriptors with ORB when using the CUDA API. I was able to do this successfully withe CPU version.
##### Steps to reproduce
``````
cv::Mat img_cpu = cv::imread("test.png", cv::IMREAD_GRAYSCALE);
cv::cuda::GpuMat img;
img.upload(img_cpu);
cv::Ptr<cv::cuda::ORB> orb =
cv::cuda::ORB::create(5000, 1.2f, 8, 31, 0, 2, 1, 31, 10, true);
cv::Ptr<cv::cuda::FastFeatureDetector> fast =
cv::cuda::FastFeatureDetector::create(100, true, 2);
cv::cuda::GpuMat keypointsGPU;
cv::cuda::GpuMat descriptorsGPU;
// this works
orb->detectAsync(img, keypointsGPU);
// but this causes segmentation fault, the same approach works without a problem in cpu
// fast->detectAsync(img, keypointsGPU);
orb->computeAsync(img, keypointsGPU, descriptorsGPU);
``````
|
bug,category: features2d,category: gpu/cuda (contrib)
|
low
|
Major
|
242,611,840 |
vue-element-admin
|
开启Vuex的严格模式会报错
|
开启严格模式会控制台报两个错,一直不知道怎么调试定位具体问题;
另外,store的app在对visitedViews进行push时,也报错,好像是堆栈溢出。
const store = new Vuex.Store({
**strict:true,**
modules: {
app,
user,
permission
},
getters
});
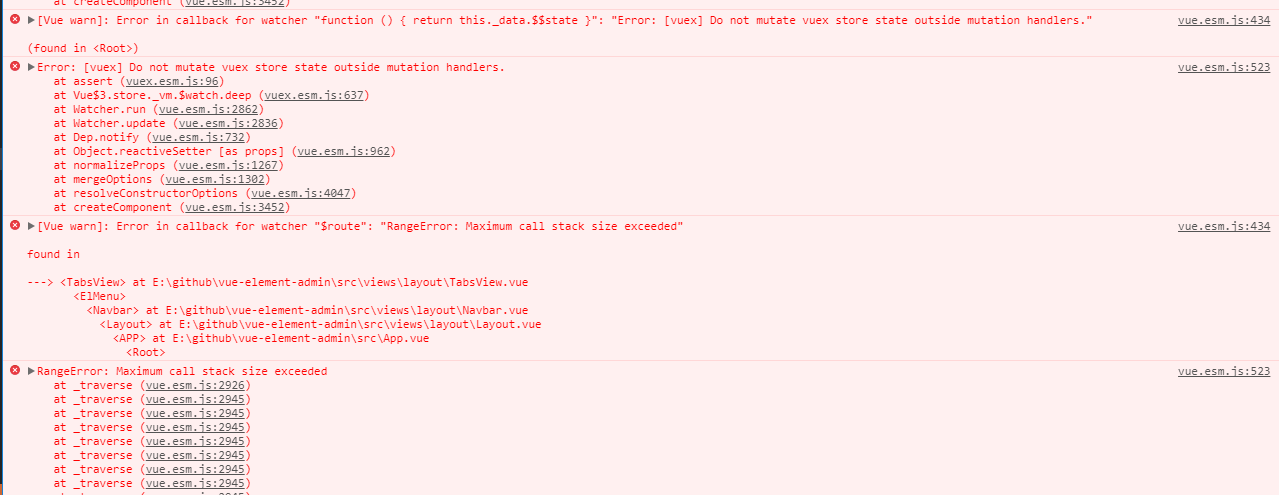
|
bug,enhancement :star:
|
medium
|
Major
|
242,620,430 |
opencv
|
Some classes which are missing in the Java wrapper
|
<!--
If you have a question rather than reporting a bug please go to http://answers.opencv.org where you get much faster responses.
If you need further assistance please read [How To Contribute](https://github.com/opencv/opencv/wiki/How_to_contribute).
This is a template helping you to create an issue which can be processed as quickly as possible. This is the bug reporting section for the OpenCV library.
-->
##### System information (version)
<!-- Example
- OpenCV => 3.1
- Operating System / Platform => Windows 64 Bit
- Compiler => Visual Studio 2015
-->
- OpenCV => 3.3.0-rc
- Operating System / Platform => Windows 64 Bit
- Compiler => Visual Studio 2017
##### Detailed description
The classes that are missing in the Java wrapper are as below:
1. **SimpleBlobDetector** and **KeyPointsFilter** classes are missing in feature2d module.
2. **LDA** (Linear Discriminant Analysis) and **SVD** (Singular Value Decomposition) classes are missing in core module
3. The **UMat** object is also missing in the Java wrapper.
_Note: The above-missed classes are what I have found till now, maybe there are more APIs which are missing in the Java wrapper. There are a lot of projects which we needed for these APIs._
**And also** if it is possible please add the documentation for the API in the Java wrapper which can help to learn and see the parameters, methods and classes description inside the IDE instead of going to OpenCV home page and search for some description of the functions are the parameters.
Big Thanks to OpenCV Team!!!
|
feature,category: java bindings
|
low
|
Critical
|
242,674,391 |
youtube-dl
|
TypeError: Struct() argument 1 must be string, not unicode
|
## Please follow the guide below
- You will be asked some questions and requested to provide some information, please read them **carefully** and answer honestly
- Put an `x` into all the boxes [ ] relevant to your *issue* (like that [x])
- Use *Preview* tab to see how your issue will actually look like
---
### Make sure you are using the *latest* version: run `youtube-dl --version` and ensure your version is *2017.07.09*. If it's not read [this FAQ entry](https://github.com/rg3/youtube-dl/blob/master/README.md#how-do-i-update-youtube-dl) and update. Issues with outdated version will be rejected.
- [x] I've **verified** and **I assure** that I'm running youtube-dl **2017.07.09**
### Before submitting an *issue* make sure you have:
- [x] At least skimmed through [README](https://github.com/rg3/youtube-dl/blob/master/README.md) and **most notably** [FAQ](https://github.com/rg3/youtube-dl#faq) and [BUGS](https://github.com/rg3/youtube-dl#bugs) sections
- [x] [Searched](https://github.com/rg3/youtube-dl/search?type=Issues) the bugtracker for similar issues including closed ones
### What is the purpose of your *issue*?
- [x] Bug report (encountered problems with youtube-dl)
- [ ] Site support request (request for adding support for a new site)
- [ ] Feature request (request for a new functionality)
- [ ] Question
- [ ] Other
---
### The following sections concretize particular purposed issues, you can erase any section (the contents between triple ---) not applicable to your *issue*
---
### If the purpose of this *issue* is a *bug report*, *site support request* or you are not completely sure provide the full verbose output as follows:
Add `-v` flag to **your command line** you run youtube-dl with, copy the **whole** output and insert it here. It should look similar to one below (replace it with **your** log inserted between triple ```):
```
import youtube_dl
File "/usr/lib/python2.7/site-packages/youtube_dl/__init__.py", line 15, in <module>
from .options import (
File "/usr/lib/python2.7/site-packages/youtube_dl/options.py", line 8, in <module>
from .downloader.external import list_external_downloaders
File "/usr/lib/python2.7/site-packages/youtube_dl/downloader/__init__.py", line 3, in <module>
from .common import FileDownloader
File "/usr/lib/python2.7/site-packages/youtube_dl/downloader/common.py", line 10, in <module>
from ..utils import (
File "/usr/lib/python2.7/site-packages/youtube_dl/utils.py", line 65, in <module>
from .socks import (
File "/usr/lib/python2.7/site-packages/youtube_dl/socks.py", line 29, in <module>
SOCKS4_DEFAULT_DSTIP = compat_struct_pack('!BBBB', 0, 0, 0, 0xFF)
TypeError: Struct() argument 1 must be string, not unicode
```
---
### Description of your *issue*, suggested solution and other information
I tried to embed youtube-dl in my code. But got this error `TypeError: Struct() argument 1 must be string, not unicode`.
This error found in python **2.7.5**. I run the script in python **2.7.9**, it's no problem, it works fine.
I think it's about string vs unicode type.
For other information, maybe this is related to this issue https://stackoverflow.com/questions/26008272/string-vs-unicode-encoding-struct-argument
Thank you
|
cant-reproduce
|
low
|
Critical
|
242,711,756 |
opencv
|
weighted centroid in phase Correlation is a reason for wrong result
|
I know that this sound like asking for a feature but it is not
it is about applying the weighted centroid as a last step in phaseCorrelate , i guess it was meant for getting sub pixel accuracy and it usually work but in my case it was a reason for wrong result
the ground truth in my case was a shift of ~ 0.5 pixel so i was expecting something between 0 and 1 but i was getting 8
with some debugging i found out that **the maximum peak location was actually 1 ("as it suppose to be ") but when applying the weighted weightedCentroid i got the (8)**
i think the reason for that is having **negative values in the Cross-correlation matrix where weighted centroid does not make sense any more**
i suggest as a small fix to add a parameter to give the option of not using the weighted centroid and returning the peak location , and again this not about extra feature , it is about a code that is causing wrong result
Best Regards
Sabaa
|
feature,category: imgproc
|
low
|
Critical
|
242,731,646 |
react-native
|
TypeError: global.nativeTraceBeginSection is not a function (Systrace)
|
### Is this a bug report?
Yes
### Have you read the Bugs section of the Contributing to React Native Guide?
Yes
### Environment
1. `react-native -v`: react-native-cli: 2.0.1, react-native: 0.46.1
2. `node -v`: v7.10.0
3. `npm -v`: 4.6.1
4. `yarn --version` (if you use Yarn): Not used in this bug
Then, specify:
- Target Platform: iOS
- Development Operating System: macOS Sierra v 10.12
- Build tools: Xcode Version 8.3.3 (8E3004b)
I'm running `react-native run-ios` deploying to iOS emulator Version 10.0 (SimulatorApp-745.10), running iOS 10.3 in an iphone 6.
### Steps to Reproduce
(Write your steps here:)
1. Run `react-native run-ios` with Systrace enabled
<img width="375" alt="dev_menu" src="https://user-images.githubusercontent.com/11222413/28170878-0d1b6aaa-67bd-11e7-9fd5-5ac653e9e00e.png">
2. Reload your app (manually or with live/hot reload)
3. Check the logs
### Expected Behavior
The app should reload normally.
### Actual Behavior
The error `TypeError:global.nativeTraceBeginSection` gets launched into the console:
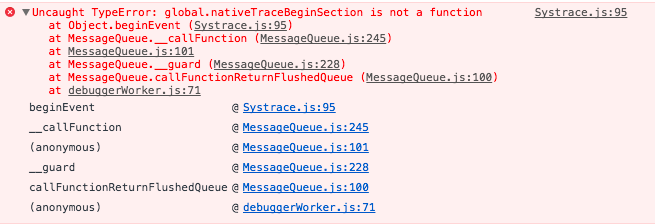
And in the UI:
<img width="375" alt="ui_error" src="https://user-images.githubusercontent.com/11222413/28171066-ad90d18c-67bd-11e7-92e3-61c394823f27.png">
After that, the whole emulator crashes and the only way to restore it is running `react-native run-ios` again.
One temporary **workaround** to get rid of this error is disabling `Systrace` in the DevMenu, but this is less than ideal.
### Reproducible Demo
https://snack.expo.io/ryCtYZHrZ
**Observation:** Even though I included a snack, I'm afraid you can't fully reproduce this problem without a Mac and an iOS emulator, the reason being that the `Systrace` is not an Expo function.
|
Bug
|
high
|
Critical
|
242,824,129 |
flutter
|
Consider not pushing intermediate routes when pushing an initial route with hierarchical structure.
|
Currently pushing an initial route with hierarchical structure implies a push for each part of the route name. E.g. having an initial route named `'/a/b'` implies pushes of `'/'`, `'/a'`, `'/a/b`. This is the behavior when navigating in directory structures but maybe not the intuitiv behavior for app navigation? Usually navigating up on a phone, means navigating in reverse chronological order, through the history of screens that the user has recently seen, as is the case when pressing back in a browser. Hence, I think we should reconsider these implicit pushes.
Any thoughts @Hixie, @collinjackson, @jakobr-google ?
|
framework,f: routes,c: proposal,P2,team-framework,triaged-framework
|
low
|
Major
|
242,850,116 |
go
|
cmd/asm, runtime: textflag for CABI
|
The runtime assembly code includes many functions that are called from outside the runtime and are called with the C ABI. This means the functions first have to save callee-saved registers manually.
For example, see runtime·sigtramp in sys_linux_*.s.
Once after diagnosing a callee-save error, I failed to save all the callee-save registers. This meant months later, @aclements had to go through the same long debugging session. We could make this more robust (and potentially make our assembly a bit easier to read) by adding a CABI text flag, and have the assembler insert code for saving and restoring registers.
cc @ianlancetaylor
|
help wanted,NeedsFix
|
low
|
Critical
|
242,851,825 |
kubernetes
|
emptyDir with medium: Memory mounts a tmpfs volume without nosuid,nodev,noexec
|
<!-- This form is for bug reports and feature requests ONLY!
If you're looking for help check [Stack Overflow](https://stackoverflow.com/questions/tagged/kubernetes) and the [troubleshooting guide](https://kubernetes.io/docs/tasks/debug-application-cluster/troubleshooting/).
-->
**Is this a BUG REPORT or FEATURE REQUEST?**:
> Uncomment only one, leave it on its own line:
>
/kind bug
> /kind feature
**What happened**:
```bash
$ kubectl exec -ti demo-1986931840-cxt6m -- sh
/ # mount | grep tmpfs
tmpfs on /dev type tmpfs (rw,nosuid,mode=755)
tmpfs on /sys/fs/cgroup type tmpfs (ro,nosuid,nodev,noexec,relatime,mode=755)
tmpfs on /tmp type tmpfs (rw,relatime) # mounted using emptyDir
tmpfs on /var/run type tmpfs (rw,relatime) # mounted using emptyDir
tmpfs on /var/run/secrets/kubernetes.io/serviceaccount type tmpfs (ro,relatime)
shm on /dev/shm type tmpfs (rw,nosuid,nodev,noexec,relatime,size=65536k)
tmpfs on /proc/kcore type tmpfs (rw,nosuid,mode=755)
tmpfs on /proc/timer_stats type tmpfs (rw,nosuid,mode=755)
```
**What you expected to happen**:
```bash
$ docker run --rm --read-only --tmpfs /tmp debian:9 mount | grep "nosuid,nodev,noexec" | grep tmpfs
tmpfs on /sys/fs/cgroup type tmpfs (ro,nosuid,nodev,noexec,relatime,mode=755)
tmpfs on /tmp type tmpfs (rw,nosuid,nodev,noexec,relatime)
shm on /dev/shm type tmpfs (rw,nosuid,nodev,noexec,relatime,size=65536k)
```
**How to reproduce it (as minimally and precisely as possible)**:
**Anything else we need to know?**:
It is recommended to mount tmpfs with `nosuid,noexec,nodev` options.
**Environment**:
- Kubernetes version (use `kubectl version`): Client Version: v1.7.0 Server Version: v1.6.4
- Cloud provider or hardware configuration**: minikube
- OS (e.g. from /etc/os-release):
- Kernel (e.g. `uname -a`):
- Install tools:
- Others:
|
kind/bug,area/security,sig/storage,kind/feature,lifecycle/frozen
|
high
|
Critical
|
242,872,595 |
TypeScript
|
Add pure and immutable keywords to ensure code has no unintended side-effects
|
The aim of this proposal is to add some immutability and pure checking into the typescript compiler. The proposal adds two new keywords that would give developers a means to define functions that are pure - meaning that the function has no-side effects, and define variables that are immutable - meaning that they can never be used in an impure context.
### Pure
The `pure` keyword is used to define a function with no side-effects ([it is allowed in the same places as the `async` keyword](https://tc39.github.io/ecma262/#sec-async-function-definitions)).
```TS
pure function x(arg) {
return arg
}
```
The keyword should be not be emitted into compiled javascript code.
A pure function:
- May not call an function not tagged as pure in the context of arguments, or this.
- `this.nonPure()`, `arg.nonPure()`, `nonPure(arg)`, and `nonPure(this)` are all disallowed.
- May make non-pure calls on instance variables, as the non-pureness applies to instance variables, so the function is technically still side-effect free:
```JS
pure function x() {
const arr = []
arr.push(1)
return arr
}
```
- - _this one i'm not entirely sure about, but it seems like it would be very hard to build pure functions without it._
- - _languages like elm get around this by having a push function which returns a new array._
- Modify the input variables
- `arg.x = 1` is disallowed within the function body.
- Modify variables on `this`
- `this.y = 1` is disallowed within the function body.
- Must return a value (otherwise there's no point to the function!).
### Immutable
Similarly a variable may be tagged as immutable:
`immutable x = []` (maybe keyword should be shortened to `immut`, or the `pure` keyword could be reused for consistency?).
This keyword is replaced with `const` in emitted code.
An immutable variable:
- Is treated as if it were `const` (i.e. its reference may not be reassigned).
- May not have any impure instance methods called on it.
- `x.nonPure()` is disallowed.
- May not be passed as an argument to impure functions.
- `nonPure(x)` is disallowed.
- May not be reassigned to a variable reference that is also not marked as pure.
- `const y = x;` is disallowed.
- `const y = { z: x }` is disallowed.
- May not have instance properties set on it.
- `x.foo = 1` is disallowed.
- May be passed to pure functions.
- `pureFn(x)` is allowed.
- May have pure instance methods called on it.
- `x.toString()` is allowed.
- For arrays, its type is strictly set at definition time, meaning that element-wise type checks will pass (fixes: https://github.com/Microsoft/TypeScript/issues/16389)
- i.e. the following code will now pass
```TS
pure function fn(arg : ('a' | 'b')[]) { }
immutable x = ['a', 'b']
fn(x)
```
### With objects/interfaces
The keyword(s) should also be allowed in `object` (and by extension `interface`) definitions:
```TS
const obj1 = {
// immutable and non-pure
immutable fn1: function () { },
// mutable and pure
fn2: pure function () { },
// immutable and pure
immutable fn3: pure function () { },
// immutable and non-pure
immutable fn3() { },
// immutable and pure
pure fn3() { },
}
interface IFace {
pure toString() : string // pure must always return a value
immutable prop : number
immutable pure frozenFn() : boolean
}
```
### With existing typings
With this proposal, the base javascript typings could be updated to support it.
I.e. the array interface would become:
```TS
interface Array<T> {
pure toString(): string;
pure toLocaleString(): string;
pure concat(...items: T[][]): T[];
pure concat(...items: (T | T[])[]): T[];
pure join(separator?: string): string;
pure indexOf(searchElement: T, fromIndex?: number): number;
pure lastIndexOf(searchElement: T, fromIndex?: number): number;
pure every(callbackfn: (this: void, value: T, index: number, array: T[]) => boolean): boolean;
pure every(callbackfn: (this: void, value: T, index: number, array: T[]) => boolean, thisArg: undefined): boolean;
pure every<Z>(callbackfn: (this: Z, value: T, index: number, array: T[]) => boolean, thisArg: Z): boolean;
pure some(callbackfn: (this: void, value: T, index: number, array: T[]) => boolean): boolean;
pure some(callbackfn: (this: void, value: T, index: number, array: T[]) => boolean, thisArg: undefined): boolean;
pure some<Z>(callbackfn: (this: Z, value: T, index: number, array: T[]) => boolean, thisArg: Z): boolean;
pure forEach(callbackfn: (this: void, value: T, index: number, array: T[]) => void): void;
pure forEach(callbackfn: (this: void, value: T, index: number, array: T[]) => void, thisArg: undefined): void;
pure forEach<Z>(callbackfn: (this: Z, value: T, index: number, array: T[]) => void, thisArg: Z): void;
pure map<U>(this: [T, T, T, T, T], callbackfn: (this: void, value: T, index: number, array: T[]) => U): [U, U, U, U, U];
pure map<U>(this: [T, T, T, T, T], callbackfn: (this: void, value: T, index: number, array: T[]) => U, thisArg: undefined): [U, U, U, U, U];
pure map<Z, U>(this: [T, T, T, T, T], callbackfn: (this: Z, value: T, index: number, array: T[]) => U, thisArg: Z): [U, U, U, U, U];
pure map<U>(this: [T, T, T, T], callbackfn: (this: void, value: T, index: number, array: T[]) => U): [U, U, U, U];
pure map<U>(this: [T, T, T, T], callbackfn: (this: void, value: T, index: number, array: T[]) => U, thisArg: undefined): [U, U, U, U];
pure map<Z, U>(this: [T, T, T, T], callbackfn: (this: Z, value: T, index: number, array: T[]) => U, thisArg: Z): [U, U, U, U];
pure map<U>(this: [T, T, T], callbackfn: (this: void, value: T, index: number, array: T[]) => U): [U, U, U];
pure map<U>(this: [T, T, T], callbackfn: (this: void, value: T, index: number, array: T[]) => U, thisArg: undefined): [U, U, U];
pure map<Z, U>(this: [T, T, T], callbackfn: (this: Z, value: T, index: number, array: T[]) => U, thisArg: Z): [U, U, U];
pure map<U>(this: [T, T], callbackfn: (this: void, value: T, index: number, array: T[]) => U): [U, U];
pure map<U>(this: [T, T], callbackfn: (this: void, value: T, index: number, array: T[]) => U, thisArg: undefined): [U, U];
pure map<Z, U>(this: [T, T], callbackfn: (this: Z, value: T, index: number, array: T[]) => U, thisArg: Z): [U, U];
pure map<U>(callbackfn: (this: void, value: T, index: number, array: T[]) => U): U[];
pure map<U>(callbackfn: (this: void, value: T, index: number, array: T[]) => U, thisArg: undefined): U[];
pure map<Z, U>(callbackfn: (this: Z, value: T, index: number, array: T[]) => U, thisArg: Z): U[];
pure filter(callbackfn: (this: void, value: T, index: number, array: T[]) => any): T[];
pure filter(callbackfn: (this: void, value: T, index: number, array: T[]) => any, thisArg: undefined): T[];
pure filter<Z>(callbackfn: (this: Z, value: T, index: number, array: T[]) => any, thisArg: Z): T[];
pure reduce(callbackfn: (previousValue: T, currentValue: T, currentIndex: number, array: T[]) => T, initialValue?: T): T;
pure reduce<U>(callbackfn: (previousValue: U, currentValue: T, currentIndex: number, array: T[]) => U, initialValue: U): U;
pure reduceRight(callbackfn: (previousValue: T, currentValue: T, currentIndex: number, array: T[]) => T, initialValue?: T): T;
pure reduceRight<U>(callbackfn: (previousValue: U, currentValue: T, currentIndex: number, array: T[]) => U, initialValue: U): U;
push(...items: T[]): number;
pop(): T | undefined;
reverse(): T[];
shift(): T | undefined;
slice(start?: number, end?: number): T[];
sort(compareFn?: (a: T, b: T) => number): this;
splice(start: number, deleteCount?: number): T[];
splice(start: number, deleteCount: number, ...items: T[]): T[];
unshift(...items: T[]): number;
[n: number]: T;
}
```
|
Suggestion,In Discussion
|
high
|
Critical
|
243,028,090 |
TypeScript
|
Multiple UMD typings in one file.d.ts
|
**TypeScript Version:** 2.4.1
**[1]** Simplistic scenario: I am Facebook and I want to ship typings for both `@types/react` and `@types/react-dom` in one file.
Currently those are 2 files, and they use UMD typings with `export as namespace`. You can:
* either move them to be non-script module-only with multiple `declare module "react"`, `declare module "react-dom"`;
* or make them both scripts with `declare namespace React`, `declare namespace ReactDOM` inside.
* * *
**[2]** Another more realistic scenario: plugins/extensions.
I am GitHub and I want to enable extensions to my shiny new GitHub Desktop (Electron) app. I want to ship a bundled `EverythingYouNeed.d.ts` file, embedding typings for the specific React version, for the specific RX version, and of course GitHub-specific APIs and extension points' typings.
At runtime I load React, ReactDOM, RX and bunch of GitHub APIs on global — so technically I can manually edit 3rd party typings from UMD style to namespaced style, before bundling them together. Extensions will just take everything off global. But it's a lot of fiddly work **over external assets (DTS)**. Maintenance cost is high.
* * *
**[3]** My actual realistic scenario is similar to GitHub one above, but in a corporate environment. I am preparing a tooling for the individual departments to use as a base for creating HTML apps. I want it to be as bundled and blackboxed as possible. Specific 3rd party libraries, specific versions etc.
As much as I can bundle JS, HTML and CSS and even compress, currently I am forced either to distribute a large number of individual decl.d.ts, or hand-edit those to allow bundling together.
For the interest of disclosure, currently I partially process external DTS files with RegExes, and partially manually edit to fit them in the bundle. Quite embarrassing!
Suggestion
=======
Two things:
* Export specific module as a specific namespace
* Module-aware syntax in non-module files
Exporting specific module as namespace
-------------
**react-bundled.d.ts**
```typescript
declare module "react" {
// declarations for React stuff...
}
export module "react" as namespace React; <-- see export specific module
declare module "react-dom" {
// declarations for ReactDOM stuff...
}
export module "react-dom" as namespace ReactDOM; <-- see export specific module
```
Module-aware syntax in scripts
------------
**react-bundle-maker.ts**
```typescript
declare import * as React from "react";
declare import * as ReactDOM from "react-dom";
```
I tell the compiler: consider typings for module X imported by the external forces. Now let me just use it.
And if I run that `react-bundle-maker.ts` file from above through `tsc --declaration`, I expect to get the same output as `react-bundled.d.ts` above.
Why these suggestions
============
These two suggestions (with possible tweaks etc.) avoid dilemmas and problems of various kinds of loaders and bundlers as discussed in [4433 Bundling TS module type definitions](https://github.com/Microsoft/TypeScript/issues/4433), [6319 Support 'target':'es5' with 'module':'es6'](https://github.com/Microsoft/TypeScript/issues/6319) and [4434 Bundling TS modules](https://github.com/Microsoft/TypeScript/issues/4434).
External loaders are dealing OK with runtime loading, but TS needs to improve its story on compile-time with modules — particularly in case of bundling for a large app scenario.
One specific hole I didn't want to dig is specifying the 'root' module for bundling. Ability to use `declare import` in scripts solves that neatly.
If we allow features I suggested, or something other to that effect, we'll have much smoother bundling workflow for DTS files.
|
Suggestion,Awaiting More Feedback
|
medium
|
Major
|
243,113,705 |
rust
|
Extend stack probe support to non-tier-1 platforms, and clarify policy for mitigating LLVM-dependent unsafety
|
As of https://github.com/rust-lang/rust/pull/42816 , all (current) tier-1 platforms are protected against the potential memory unsafety demonstrated in https://github.com/rust-lang/rust/issues/16012 . However, this protection is dependent upon platform-dependent LLVM support, so AFAIK most of our supported tier-2 and tier-3 platforms ( https://forge.rust-lang.org/platform-support.html ) are still theoretically unprotected.
Proper resolution of this issue will depend on extending LLVM's stack probe support for various platforms, (which should be done regardless of the discussion in the next paragraph). See https://reviews.llvm.org/D34387 for pcwalton's original x86 implementation, https://reviews.llvm.org/D34386 for the implementation of the attribute itself, and https://github.com/rust-lang/rust/pull/42816 for the work needed on rustc's side.
But here's the more important concern: the list of potential platforms is unbounded, and we need to decide where to draw the line. As minor as the safety hole may be, is it an abrogation of Rust's claim to guarantee memory safety if that safety is only enforced on "popular" platforms? If so, is it worth considering some sort of blanket check that can be implemented in the frontend for use only on platforms where stack probes are unimplemented? For instance, given that we statically know the stack size of all types, could we perhaps enforce an upper bound on the size of a type (perhaps only in debug mode)? I think this discussion may be relevant to https://github.com/rust-lang/rust/issues/10184 as well.
|
A-LLVM,C-enhancement,A-codegen,P-medium,T-compiler,I-unsound,E-needs-mcve,A-stack-probe
|
medium
|
Critical
|
243,134,988 |
rust
|
Tracking issue for Vec::extract_if and LinkedList::extract_if
|
Feature gate: `#![feature(extract_if)]` (previously `drain_filter`)
This is a tracking issue for `Vec::extract_if` and `LinkedList::extract_if`, which can be used for random deletes using iterators.
### Public API
```rust
pub mod alloc {
pub mod vec {
impl<T, A: Allocator> Vec<T, A> {
pub fn extract_if<F>(&mut self, filter: F) -> ExtractIf<'_, T, F, A>
where
F: FnMut(&mut T) -> bool,
{
}
}
#[derive(Debug)]
pub struct ExtractIf<'a, T, F, #[unstable(feature = "allocator_api", issue = "32838")] A: Allocator = Global>
where
F: FnMut(&mut T) -> bool, {}
impl<T, F, A: Allocator> Iterator for ExtractIf<'_, T, F, A>
where
F: FnMut(&mut T) -> bool,
{
type Item = T;
fn next(&mut self) -> Option<T> {}
fn size_hint(&self) -> (usize, Option<usize>) {}
}
impl<T, F, A: Allocator> Drop for ExtractIf<'_, T, F, A>
where
F: FnMut(&mut T) -> bool,
{
fn drop(&mut self) {}
}
}
pub mod collections {
pub mod linked_list {
impl<T> LinkedList<T> {
pub fn extract_if<F>(&mut self, filter: F) -> ExtractIf<'_, T, F>
where
F: FnMut(&mut T) -> bool,
{
}
}
pub struct ExtractIf<'a, T: 'a, F: 'a>
where
F: FnMut(&mut T) -> bool, {}
impl<T, F> Iterator for ExtractIf<'_, T, F>
where
F: FnMut(&mut T) -> bool,
{
type Item = T;
fn next(&mut self) -> Option<T> {}
fn size_hint(&self) -> (usize, Option<usize>) {}
}
impl<T, F> Drop for ExtractIf<'_, T, F>
where
F: FnMut(&mut T) -> bool,
{
fn drop(&mut self) {}
}
impl<T: fmt::Debug, F> fmt::Debug for ExtractIf<'_, T, F>
where
F: FnMut(&mut T) -> bool,
{
fn fmt(&self, f: &mut fmt::Formatter<'_>) -> fmt::Result {}
}
}
}
}
```
### Steps / History
- [x] Implementation: #43245
- [x] removed drain-on-drop behavior, renamed to extract_if
- #104455
- https://github.com/rust-lang/libs-team/issues/136
- [ ] Stabilization PR
### Unresolved Questions
- What should the method be named?
- Should `extract_if` accept a `Range` argument?
- Missing `Send`+`Sync` impls on linked list's ExtractIf, [see comment](https://github.com/rust-lang/rust/issues/43244#issuecomment-1268857915)
See https://github.com/rust-lang/rust/issues/43244#issuecomment-641638196 for a more detailed summary of open issues.
|
A-collections,T-libs-api,B-unstable,C-tracking-issue,disposition-merge,finished-final-comment-period,Libs-Tracked
|
high
|
Critical
|
243,138,954 |
TypeScript
|
Type-check jsdoc-annotated property assignments
|
**TypeScript Version:** nightly (2.5.0-dev.20170712)
**Code**
```ts
// @ts-check
/** @type {string} */
exports.x = 1;
```
**Expected behavior:**
Error.
**Actual behavior:**
No error.
And if I import `x` in another module, it is typed as `string`.
|
Bug,Domain: JSDoc,Domain: JavaScript
|
low
|
Critical
|
243,169,671 |
rust
|
Coroutine tracing of borrows is quite conservative
|
This example really should work, but does not:
```rust
fn yield_during_range_iter() {
// Should be OK.
let mut b = || {
let v = vec![1,2,3];
for i in 0..v.len() {
yield v[i];
}
};
b.resume();
}
fn main() { }
```
I get:
```
error[E0624]: borrow may still be in use when generator yields
--> /home/nmatsakis/versioned/rust-1/src/test/ui/generator/yield-while-iterating.rs:75:21
|
75 | for i in 0..v.len() {
| ^
76 | yield v[i];
| ---------- possible yield occurs here
error[E0624]: borrow may still be in use when generator yields
--> /home/nmatsakis/versioned/rust-1/src/test/ui/generator/yield-while-iterating.rs:76:19
|
76 | yield v[i];
| ------^--- possible yield occurs here
```
I think the problem is that the current code for deciding which temporaries are in scope is quite overly conservative. (On a related note, we also have to unconditionally throw booleans into the mix because of drop flags.)
cc @Zoxc @alexcrichton
|
C-enhancement,T-compiler,A-coroutines,F-coroutines
|
low
|
Critical
|
243,176,394 |
youtube-dl
|
Add support for videos saved with Internet Archive Wayback Machine
|
## Please follow the guide below
- You will be asked some questions and requested to provide some information, please read them **carefully** and answer honestly
- Put an `x` into all the boxes [ ] relevant to your *issue* (like that [x])
- Use *Preview* tab to see how your issue will actually look like
---
### Make sure you are using the *latest* version: run `youtube-dl --version` and ensure your version is *2017.07.15*. If it's not read [this FAQ entry](https://github.com/rg3/youtube-dl/blob/master/README.md#how-do-i-update-youtube-dl) and update. Issues with outdated version will be rejected.
- [x] I've **verified** and **I assure** that I'm running youtube-dl **2017.07.15**
### What is the purpose of your *issue*?
- [ ] Bug report (encountered problems with youtube-dl)
- [x] Site support request (request for adding support for a new site)
- [ ] Feature request (request for a new functionality)
- [ ] Question
- [ ] Other
---
Heres some examples of URL's saved with wayback:
https://web.archive.org/web/20150517183426/https://www.youtube.com/watch?v=V46KYxFav24
https://web.archive.org/web/20071015051038/http://youtube.com:80/watch?v=JdLCEwEFCMU
https://web.archive.org/web/20110531000748/http://www.youtube.com/watch?v=bVUnpkvgHaw&gl=US&hl=en&has_verified=1
|
site-support-request
|
medium
|
Critical
|
243,195,708 |
youtube-dl
|
Problems on some techtalks.tv videos (but not all)
|
- [X] I've **verified** and **I assure** that I'm running youtube-dl **2017.07.15**
### Before submitting an *issue* make sure you have:
- [X] At least skimmed through [README](https://github.com/rg3/youtube-dl/blob/master/README.md) and **most notably** [FAQ](https://github.com/rg3/youtube-dl#faq) and [BUGS](https://github.com/rg3/youtube-dl#bugs) sections
- [X] [Searched](https://github.com/rg3/youtube-dl/search?type=Issues) the bugtracker for similar issues including closed ones
### What is the purpose of your *issue*?
- [X] Bug report (encountered problems with youtube-dl)
- [ ] Site support request (request for adding support for a new site)
- [ ] Feature request (request for a new functionality)
- [ ] Question
- [ ] Other
---
### If the purpose of this *issue* is a *bug report*, *site support request* or you are not completely sure provide the full verbose output:
```
$ youtube-dl -v http://techtalks.tv/talks/memory-networks-for-language-understanding/62356/
[debug] System config: []
[debug] User config: []
[debug] Custom config: []
[debug] Command-line args: [u'-v', u'http://techtalks.tv/talks/memory-networks-for-language-understanding/62356/']
[debug] Encodings: locale UTF-8, fs utf-8, out UTF-8, pref UTF-8
[debug] youtube-dl version 2017.07.15
[debug] Python version 2.7.13 - Darwin-16.6.0-x86_64-i386-64bit
[debug] exe versions: avconv 12, avprobe 12, ffmpeg 3.3.2, ffprobe 3.3.2, rtmpdump 2.4
[debug] Proxy map: {}
[TechTalks] 62356: Downloading webpage
[debug] Invoking downloader on u'rtmp://webcast.weyond.com/vod'
[download] Destination: Memory Networks for Language Understanding-62356.flv
[debug] rtmpdump command line: rtmpdump --verbose -r 'rtmp://webcast.weyond.com/vod' -o 'Memory Networks for Language Understanding-62356.flv.part' --playpath 'icml/2016/tutorials/mp4:tuts-crown-2' --resume --skip 1
[rtmpdump] RTMPDump v2.4
[rtmpdump] (c) 2010 Andrej Stepanchuk, Howard Chu, The Flvstreamer Team; license: GPL
[rtmpdump] DEBUG: Parsing...
[rtmpdump] DEBUG: Parsed protocol: 0
[rtmpdump] DEBUG: Parsed host : webcast.weyond.com
[rtmpdump] DEBUG: Parsed app : vod
[rtmpdump] DEBUG: Number of skipped key frames for resume: 1
[rtmpdump] DEBUG: Protocol : RTMP
[rtmpdump] DEBUG: Hostname : webcast.weyond.com
[rtmpdump] DEBUG: Port : 1935
[rtmpdump] DEBUG: Playpath : icml/2016/tutorials/mp4:tuts-crown-2
[rtmpdump] DEBUG: tcUrl : rtmp://webcast.weyond.com:1935/vod
[rtmpdump] DEBUG: app : vod
[rtmpdump] DEBUG: live : no
[rtmpdump] DEBUG: timeout : 30 sec
[rtmpdump] DEBUG: Failed to get last keyframe.
[rtmpdump] DEBUG: Closing connection.
[rtmpdump] 0 bytes
[rtmpdump] RTMPDump v2.4
[rtmpdump] (c) 2010 Andrej Stepanchuk, Howard Chu, The Flvstreamer Team; license: GPL
[rtmpdump] DEBUG: Parsing...
[rtmpdump] DEBUG: Parsed protocol: 0
[rtmpdump] DEBUG: Parsed host : webcast.weyond.com
[rtmpdump] DEBUG: Parsed app : vod
[rtmpdump] DEBUG: Number of skipped key frames for resume: 1
[rtmpdump] DEBUG: Number of skipped key frames for resume: 1
[rtmpdump] DEBUG: Protocol : RTMP
[rtmpdump] DEBUG: Hostname : webcast.weyond.com
[rtmpdump] DEBUG: Port : 1935
[rtmpdump] DEBUG: Playpath : icml/2016/tutorials/mp4:tuts-crown-2
[rtmpdump] DEBUG: tcUrl : rtmp://webcast.weyond.com:1935/vod
[rtmpdump] DEBUG: app : vod
[rtmpdump] DEBUG: live : no
[rtmpdump] DEBUG: timeout : 30 sec
[rtmpdump] DEBUG: Failed to get last keyframe.
[rtmpdump] DEBUG: Closing connection.
ERROR: rtmpdump exited with code 1
File "/usr/local/Cellar/python/2.7.13/Frameworks/Python.framework/Versions/2.7/lib/python2.7/runpy.py", line 174, in _run_module_as_main
"__main__", fname, loader, pkg_name)
File "/usr/local/Cellar/python/2.7.13/Frameworks/Python.framework/Versions/2.7/lib/python2.7/runpy.py", line 72, in _run_code
exec code in run_globals
File "/usr/local/bin/youtube-dl/__main__.py", line 19, in <module>
youtube_dl.main()
File "/usr/local/bin/youtube-dl/youtube_dl/__init__.py", line 465, in main
_real_main(argv)
File "/usr/local/bin/youtube-dl/youtube_dl/__init__.py", line 455, in _real_main
retcode = ydl.download(all_urls)
File "/usr/local/bin/youtube-dl/youtube_dl/YoutubeDL.py", line 1941, in download
url, force_generic_extractor=self.params.get('force_generic_extractor', False))
File "/usr/local/bin/youtube-dl/youtube_dl/YoutubeDL.py", line 787, in extract_info
return self.process_ie_result(ie_result, download, extra_info)
File "/usr/local/bin/youtube-dl/youtube_dl/YoutubeDL.py", line 841, in process_ie_result
return self.process_video_result(ie_result, download=download)
File "/usr/local/bin/youtube-dl/youtube_dl/YoutubeDL.py", line 1584, in process_video_result
self.process_info(new_info)
File "/usr/local/bin/youtube-dl/youtube_dl/YoutubeDL.py", line 1848, in process_info
success = dl(filename, info_dict)
File "/usr/local/bin/youtube-dl/youtube_dl/YoutubeDL.py", line 1790, in dl
return fd.download(name, info)
File "/usr/local/bin/youtube-dl/youtube_dl/downloader/common.py", line 361, in download
return self.real_download(filename, info_dict)
File "/usr/local/bin/youtube-dl/youtube_dl/downloader/rtmp.py", line 202, in real_download
self.report_error('rtmpdump exited with code %d' % retval)
File "/usr/local/bin/youtube-dl/youtube_dl/downloader/common.py", line 163, in report_error
self.ydl.report_error(*args, **kargs)
File "/usr/local/bin/youtube-dl/youtube_dl/YoutubeDL.py", line 604, in report_error
self.trouble(error_message, tb)
File "/usr/local/bin/youtube-dl/youtube_dl/YoutubeDL.py", line 566, in trouble
tb_data = traceback.format_list(traceback.extract_stack())
```
---
### Description of your *issue*, suggested solution and other information
I'm trying to download a video from techtalks.tv with the above command, which fails. Looking through the existing issues related to techtalks.tv, I found [another issue](https://github.com/rg3/youtube-dl/issues/11448). The link mentioned in that issue does not fail, I can download that video without problems. So I think the problem isn't in my rtmpdump installation.
|
cant-reproduce
|
low
|
Critical
|
243,206,765 |
TypeScript
|
Support `@abstract` JSDoc tag
|
**TypeScript Version:** nightly (2.5.0-dev.20170712)
**Code**
```ts
/** @abstract */
class C {}
new C();
```
**Expected behavior:**
Error.
**Actual behavior:**
No error.
|
Suggestion,Domain: JavaScript,Experience Enhancement
|
medium
|
Critical
|
243,210,904 |
go
|
sync: reduce pointer overhead in Map
|
The Go 1.9 implementation of `sync.Map` is very pointer-heavy. That wastes a bit of CPU (following and caching those pointers and their address translations) and also consumes more steady-state memory than it potentially needs to (by at least one pointer per entry).
Now that `sync.Map` is in the standard library (instead of just `x/sync`), we can potentially make use of knowledge about the runtime implementations of `map`, `interface{}` and/or `atomic.Value` to flatten out some of those pointers.
In particular we can probably change the `readOnly.m` field to contain a `runtime.hmap` directly instead of a `map` variable that points to it.
We may also be able to change the elements of that map from `*entry` to `entry`, since their addresses will be stable (unless we address https://github.com/golang/go/issues/19094). It's not obvious to me whether that would add too much complexity to the process of promoting the read-write map to read-only (which is currently just an atomic store): in particular, we would need some way to ensure that no thread is trying to update the entries of the previous read-only map, which might require that we only promote read-write to read-only during a GC safe-point.
Finally, we may be able to store `interface{}` values directly in the `entry` struct rather than unsafe-pointers-to-interfaces, although (as `atomic.Value` demonstrates) the synchronization for updates may be very subtle.
Further pointer optimizations may be possible; those are just the ones I can think of.
----
I don't intend to do this optimization work myself any time soon, but I wanted to document this idea in case someone else in the community has a use-case that motivates them to address it.
|
Performance,compiler/runtime
|
low
|
Major
|
243,211,220 |
go
|
sync: reduce (*Map).Load penalty for Stores with new keys
|
In the Go 1.9 implementation of `sync.Map`, storing _any_ new key in the map causes `Load` calls on _all_ new keys to acquire a mutex until enough misses have occurred to promote the read-write map again.
That's probably fine for append-only maps (such as the `sync.Map` uses in the standard library), because it goes away in the steady state. However, it's problematic for use in caches, where there may be frequent `Load` and `Store` calls for keys that miss the cache.
I suspect we could substantially reduce the `Load` penalty by using a Bloom or HyperLogLog filter instead of a simple boolean to store the set of new keys: a `Load` that misses in the read-only map would still have more cache misses due to writes to the filter, but it would no longer block `Store` calls or contend with other `Load`s.
|
Performance,compiler/runtime
|
low
|
Major
|
243,212,344 |
go
|
x/mobile: Process crashed as JNI exception isn't cleaned before next call.
|
### What version of Go are you using (`go version`)?
go1.8.3
(
gomobile version +0f31740 Wed Apr 19 17:20:05 2017 +0000 (android); androidSDK=[removed]/platforms/android-26
)
### What operating system and processor architecture are you using (`go env`)?
linux/amd64
cross complied to
android/arm
### What did you do?
Calling Java function from go part of android app(with interface), and an unchecked exception was thrown at Java side.
This is the abridged version of original crash log:
```
07-15 21:00:20.352 2854-2854/? A/DEBUG: Abort message: 'art/runtime/java_vm_ext.cc:410] JNI DETECTED ERROR IN APPLICATION: JNI CallStaticVoidMethod called with pending exception java.lang.IllegalStateException: Already closed'
07-15 21:00:20.352 2854-2854/? A/DEBUG: x0 0000000000000000 x1 00000000000027c8 x2 0000000000000006 x3 0000000000000000
07-15 21:00:20.352 2854-2854/? A/DEBUG: x4 0000000000000000 x5 0000000000000001 x6 0000000000000000 x7 0000000000000000
07-15 21:00:20.352 2854-2854/? A/DEBUG: x8 0000000000000083 x9 000000000002fc80 x10 0000000001deffa0 x11 0000000001df00e0
07-15 21:00:20.352 2854-2854/? A/DEBUG: x12 0000000000000000 x13 0000007fa1344000 x14 0000000000000000 x15 0000000000000000
07-15 21:00:20.352 2854-2854/? A/DEBUG: x16 0000007fa1336568 x17 0000007fa12c92fc x18 0000007fa1347f50 x19 0000007f81433500
07-15 21:00:20.352 2854-2854/? A/DEBUG: x20 0000007f81433440 x21 000000000000000b x22 0000000000000006 x23 0000007f823f4400
07-15 21:00:20.352 2854-2854/? A/DEBUG: x24 0000007f979afc80 x25 0000000000000000 x26 0000007f9dcec000 x27 0000000000000000
07-15 21:00:20.352 2854-2854/? A/DEBUG: x28 0000007f823f4400 x29 0000007f814328e0 x30 0000007fa12c6a98
07-15 21:00:20.352 2854-2854/? A/DEBUG: sp 0000007f814328e0 pc 0000007fa12c9304 pstate 0000000020000000
07-15 21:00:20.357 2854-2854/? A/DEBUG: backtrace:
07-15 21:00:20.357 2854-2854/? A/DEBUG: #00 pc 0000000000069304 /system/lib64/libc.so (tgkill+8)
07-15 21:00:20.357 2854-2854/? A/DEBUG: #01 pc 0000000000066a94 /system/lib64/libc.so (pthread_kill+68)
07-15 21:00:20.357 2854-2854/? A/DEBUG: #02 pc 00000000000239f8 /system/lib64/libc.so (raise+28)
07-15 21:00:20.357 2854-2854/? A/DEBUG: #03 pc 000000000001e198 /system/lib64/libc.so (abort+60)
07-15 21:00:20.357 2854-2854/? A/DEBUG: #04 pc 00000000004336c0 /system/lib64/libart.so (_ZN3art7Runtime5AbortEv+324)
07-15 21:00:20.357 2854-2854/? A/DEBUG: #05 pc 0000000000136f78 /system/lib64/libart.so (_ZN3art10LogMessageD2Ev+3176)
07-15 21:00:20.357 2854-2854/? A/DEBUG: #06 pc 000000000030f248 /system/lib64/libart.so (_ZN3art9JavaVMExt8JniAbortEPKcS2_+2080)
07-15 21:00:20.357 2854-2854/? A/DEBUG: #07 pc 000000000030f6c8 /system/lib64/libart.so (_ZN3art9JavaVMExt9JniAbortVEPKcS2_St9__va_list+116)
07-15 21:00:20.357 2854-2854/? A/DEBUG: #08 pc 0000000000142d34 /system/lib64/libart.so (_ZN3art11ScopedCheck6AbortFEPKcz+144)
07-15 21:00:20.357 2854-2854/? A/DEBUG: #09 pc 0000000000149be4 /system/lib64/libart.so (_ZN3art11ScopedCheck5CheckERNS_18ScopedObjectAccessEbPKcPNS_12JniValueTypeE.constprop.116+6088)
07-15 21:00:20.358 2854-2854/? A/DEBUG: #10 pc 000000000015c8f0 /system/lib64/libart.so (_ZN3art8CheckJNI11CallMethodVEPKcP7_JNIEnvP8_jobjectP7_jclassP10_jmethodIDSt9__va_listNS_9Primitive4TypeENS_10InvokeTypeE+948)
07-15 21:00:20.358 2854-2854/? A/DEBUG: #11 pc 000000000015d72c /system/lib64/libart.so (_ZN3art8CheckJNI20CallStaticVoidMethodEP7_JNIEnvP7_jclassP10_jmethodIDz+160)
07-15 21:00:20.358 2854-2854/? A/DEBUG: #12 pc 00000000004f8038 /data/app/org.kkdev.v2raygo-1/lib/arm64/libgojni.so (go_seq_inc_ref+80)
```
I have successfully reproduced this crash. Try this binary apk(built with debug enabled):
[app.zip](https://github.com/golang/go/files/1150775/app.zip)
*Reproduced log:*
https://gist.github.com/xiaokangwang/8d7f5aefd98d7c52c644b4c815097eb5
*Reproduce code:*
Go part of reproduce
https://github.com/xiaokangwang/gomobilecrashreproducego
Java part of reproduce
https://github.com/xiaokangwang/gomobilecrashreproducejava
### What did you expect to see?
Handle this situation gracefully, for example a API for dealing with unchecked exception, it can be:
- a chan receiving unchecked exception if none is defined, the default handing comes in.
- a runtime setting for defining what to do in this situation(quit or let it go)
- a warn message at console/log if no policy is defined and an exception was thrown.
### What did you see instead?
Just crash, and destabilize entire app.
This issue also have been spotted at https://github.com/ethereum/go-ethereum/issues/14437, but seems to be ignored.
Original discovered as my app's service crash frequently and render it unusable.
|
mobile
|
low
|
Critical
|
243,214,309 |
go
|
all: add a few READMEs to help new people navigate the source tree
|
The api directory has a friendly README file in it explaining what's in it. We should add short READMEs to some of the other top-level directories, particularly doc, lib, misc, and test.
|
Documentation,NeedsFix
|
low
|
Major
|
243,216,205 |
go
|
sync: reduce contention between Map operations with new-but-disjoint keys
|
The Go 1.9 implementation of `sync.Map` uses a single `Mutex` to guard the read-write map containing new keys. That makes `Store` calls with different new keys always contend with each other, and also contend with `Load` calls with different new keys, even if the `Load`s and `Store`s for each key are confined to a single thread.
That doesn't really matter for the `sync.Map` use-cases in the standard library because they do not operate on new keys in the steady state, but it limits the utility of `sync.Map` for use-cases involving a high rate of churn. Such use-cases may include:
* caches with high eviction rates, such as caches fronting key-value storage services
* maps of RPC or HTTP stream ID to handler state
* maps of opaque handles to Go pointers in order to write C-exported APIs that comply with [cgo pointer-passing](https://golang.org/cmd/cgo/#hdr-Passing_pointers) rules
We should explore ways to address new-key contention, such as sharding the read-write maps and associated locks (as suggested in https://github.com/golang/go/issues/20360), journaling writes (and using a Bloom or HyperLogLog filter to avoid reading the journal, along the lines of https://github.com/golang/go/issues/21032), or storing the read-write map in an atomic tree data structure instead of a built-in `map`.
|
Performance,compiler/runtime
|
medium
|
Critical
|
243,224,167 |
vscode
|
Can the VSCode gutter be made smaller, i.e., decreased size/width?
|
<!-- Do you have a question? Please ask it on http://stackoverflow.com/questions/tagged/vscode. -->
<!-- Use Help > Report Issues to prefill these. -->
- VSCode Version: 1.14.1
- OS Version: Windows 10 build 15063
Comparing Sublime Text gutter with VSCode gutter, we conclude the VSCode gutter is too big.
**Sublime Text**
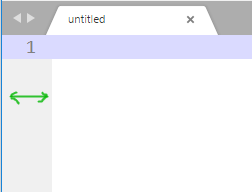
**Notepad++**
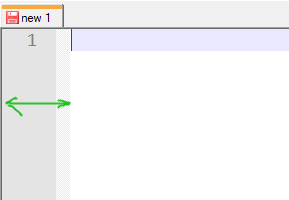
**VSCode**
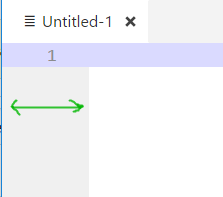
Can the VSCode gutter be made smaller, i.e., decreased size/width?
___
This question can be found on StackOverflow:
1. [How do I change the Gutter Width in VS Code?](https://stackoverflow.com/questions/43957564/how-do-i-change-the-gutter-width-in-vs-code)
|
feature-request,editor-core
|
high
|
Critical
|
243,319,417 |
go
|
cmd/go: do not ignore explicit _foo.go argument
|
```
go version go1.8.3 linux/amd64
GOPATH="/home/lion/aosc/ciel"
PWD="/home/lion/aosc/ciel"
```
### What did you do?
```
go fmt src/ciel/_main.go
go vet src/ciel/_main.go
go generate src/ciel/_main.go
...
```
### What did you expect to see?
Take `go fmt` as the example.
It should do something like `go fmt src/ciel/anotherfile.go`.
### What did you see instead?
`can't load package: package main: no buildable Go source files in /home/lion/aosc/ciel/src/ciel`
I understand `_file` will be ignored by go tools when I specified a package:
https://godoc.org/cmd/go/#hdr-Description_of_package_lists
> Directory and file names that begin with "." or "_" are ignored by the go tool, as are directories named "testdata".
But, according to the context, this rule is for package, when I pass a file name to argument explicitly, it should not ignore them.
I think this maybe relevant to `go/build.Context.UseAllFiles`.
https://godoc.org/pkg/go/build/#Context
```
type Context struct {
GOARCH string // target architecture
GOOS string // target operating system
GOROOT string // Go root
GOPATH string // Go path
CgoEnabled bool // whether cgo can be used
UseAllFiles bool // use files regardless of +build lines, file names
Compiler string // compiler to assume when computing target paths
```
|
NeedsFix
|
low
|
Minor
|
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.