id
int64 393k
2.82B
| repo
stringclasses 68
values | title
stringlengths 1
936
| body
stringlengths 0
256k
β | labels
stringlengths 2
508
| priority
stringclasses 3
values | severity
stringclasses 3
values |
---|---|---|---|---|---|---|
429,103,680 | three.js | Volumetric SpotLights | Hey all,
I came across a cool and efficient implementation of [volumetrics for spotlights](http://john-chapman-graphics.blogspot.com/2013/01/good-enough-volumetrics-for-spotlights.html) -- and was able to achieve a pretty neat effect by using this approach paired with a noise texture and using multiple cones with decreasing radii.
<img width="539" alt="Screen Shot 2019-04-04 at 1 10 29 PM" src="https://user-images.githubusercontent.com/17795014/55533610-0f3d9a00-56dc-11e9-86f8-3dd044ef189d.png">
I think this could be helpful for people, as it is the only one i've seen that does not require multiple render passes. I think it's not fundamental enough to add to the `THREE.Spotlight`, but probably better for an example.
Let me know! | Addons | low | Major |
429,106,245 | electron | Support Custom Media Stream Tracks | <!-- As an open source project with a dedicated but small maintainer team, it can sometimes take a long time for issues to be addressed so please be patient and we will get back to you as soon as we can.
-->
### Preflight Checklist
<!-- Please ensure you've completed the following steps by replacing [ ] with [x]-->
* [x] I have read the [Contributing Guidelines](https://github.com/electron/electron/blob/master/CONTRIBUTING.md) for this project.
* [x] I agree to follow the [Code of Conduct](https://github.com/electron/electron/blob/master/CODE_OF_CONDUCT.md) that this project adheres to.
* [x] I have searched the issue tracker for a feature request that matches the one I want to file, without success.
### Problem Description
<!-- Is your feature request related to a problem? Please add a clear and concise description of what the problem is. -->
We need a desktop capturer with some additional features, i.e. excluding certain windows from capture. We implemented such a capturer as a plugin. It periodically produces a bitmap, which then is drawn into a hidden canvas element by JavaScript and then a media stream for WebRTC is produced by Chromeβs canvas capturer.

This solution proved to be workable, but it also quite costly in CPU usage terms. As an experiment we produced plugin that uses internal Chromium API to copy video frames from capturer into MediaStream.

This optimization significantly reduced CPU usage (from 60% to 25% CPU usage on Windows capturing 2880x1800 desktop). But due to use of Chromeβs internal API optimized plugin requires use of component Electron build.
### Proposed Solution
<!-- Describe the solution you'd like in a clear and concise manner -->
We propose extension of Electron API intended to allow app authors to implement custom media stream tracks.
Example of proposed API use:
const { customMedia } = require("electron");
let track = customMedia.createTrack({
info: {
resolution: { width: 1920, hight: 1080 }
frameRate: 30
},
startCapture: (queue) => {
this.interval = setInterval({
let frame = queue.allocateFrame({
pixelFormat: 'YUV'
});
// frame.y, frame.u and frame.v are ArrayBuffers
// containing pixels from each color plane
frame.y.fill(76); // filling the buffer with color red
frame.u.fill(84);
frame.v.fill(255);
queue.queueFrame(Date.now(), frame);
});
},
stopCapture: () => {
clearInterval(this.interval);
}
})
let mediaStream = new MediaStream();
mediaStream.addTrack(track);
//this is a html5 video element
video.srcObject = mediaStream
**API description:**
`createTrack(source)` - creates a standard MediaStreamTrack wrapping video source provided by the client. Being a standard object it can be added to any media stream, and in the stream passed as source to an html5 video element or as source for WebRTC. Its argument is a video source object provided by API client. It should have `info` field containing metadata, `startCapture` and `stopCapture` callbacks.
`source.info` - describes video track. Sets track resolution and framerate. Those will be reported as metadata by video element for example.
`source.startCapture(queue)` - starts video source. queue object is passed to client from API to allocate and queue video frames for playback.
`source.stopCapture()` - stops video source.
`queue.allocateFrame(spec)` - allocates or reuse existing video frame for the client. spec specifies desired frame properties, for example resolution and pixel format.
`queue.queueFrame(timestamp, frame)` - queues frame for playback.
`frame` - video frame object. Should contain means to access pixel data, depending on pixel format. YUV frames should contain y,u,v properties that should be ArrayBuffers pointing to appropriate color planes.
**C++ interoperability**
To ease interoperability of this API with third party C++ plugins queue should wrap a C++ helper object.
```
enum class Plane {Y,U,V};
struct Format {...}; // pixel format info, resolution etc.
struct VideoFrame {
virtual Format format() =0;
virtual void* data(Plane plane) =0;
};
struct Timestamp {};
struct VideoFrameQueue {
virtual VideoFrame* dequeueFrame() = 0;
virtual void queueFrame(Timestamp, VideoFrame*) = 0;
};
VideoFrameQueue* unwrapQueue(v8::Handle<Value> value);
```
<!-- ### Alternatives Considered
-->
<!-- A clear and concise description of any alternative solutions or features you've considered. -->
<!-- ### Additional Information
-->
<!-- Add any other context about the problem here. -->
| enhancement :sparkles: | low | Major |
429,168,725 | react | [Concurrent] Safely disposing uncommitted objects | ## How to safely keep a reference to uncommitted objects and dispose of them on unmount?
For a MobX world, we are trying to [prepare for the Concurrent mode](https://github.com/mobxjs/mobx-react-lite/issues/53). In short, there is a [Reaction object being created](https://github.com/mobxjs/mobx-react-lite/blob/231be100e6ba458f40642adb9f0bc77aa4d366ce/src/useObserver.ts#L32
) to track for observables and it is stored within `useRef`.
The major problem is, that we can't just `useEffect` to create it in a safe way later. We need it to start tracking the observables on a first render otherwise we might miss some updates and cause inconsistent behavior.
We do have a [semi-working solution](https://github.com/mobxjs/mobx-react-lite/pull/121), basically, a custom made garbage collector based on `setTimeout`. However, it's unreliable as it can accidentally dispose of Reactions that are actually being used but weren't committed yet.
Would love to hear we are overlooking some obvious solution there. | Type: Discussion | high | Critical |
429,169,135 | vscode | [folding] Distinctly highlight corresponding `#endregion` for `#region X` comments | That would apply to all supported languages. But I considered it for Python only, and so may be missing something.
In python, standing on a `#region` marker highlights all `#region` tokens in the file, as is normal for other tokens in plain source and in comments.
**Request**: Apply a different highlighting rule (perhaps "line boxes" as is applied to matching brackets/parentheses/braces) to the `#endregion` corresponding to the `#region` under the cursor, to make clear the region's boundary. | feature-request,editor-folding | low | Minor |
429,178,980 | pytorch | More efficient STFT on CUDA | ## π Feature
This is a rather difficult project, but I wanted to put down the idea anyway, maybe someone is bold enough to take it up.
`cuFFT` allows callbacks that would allow for some interesting speedups for the STFT. The callback for loading a window could handle:
* optional conversion from int to float
* padding frames that exceed the border
* multiplication with the window function
The callback for writing the result could handle:
* optional computation of magnitudes from real and imaginary part
## Motivation
The current implementation of `torch.stft` does an explicit copy of all the signal if it must be padded, and it does an explicit expansion of all the signal if a window function must be applied. This can eat up lots of memory and time.
Similarly, many use cases will start with 16-bit signals that need to be converted to float before, and are only interested in magnitudes rather than the complex results.
## Pitch
The interface of `torch.stft` would need to be adapted to allow a dtype conversion, and to specify whether to compute the complex result or only the magnitudes. When possible, the implementation would use cuFFT callbacks to perform the operations.
## Alternatives
To reduce the impact of padding, if the signals are large enough to be worth it (or the user requests it), they could be cut into three parts: the beginning that needs to be padded, the main part that doesn't, and the end that needs to be padded. The results would be written into the correct locations in a preallocated buffer.
## Additional context
Pointers to cuFFT callbacks:
* https://devblogs.nvidia.com/cuda-pro-tip-use-cufft-callbacks-custom-data-processing/
* https://stackoverflow.com/questions/39535141/why-does-cufft-performance-suffer-with-overlapping-inputs | module: performance,feature,module: cuda,triaged | low | Major |
429,238,591 | go | x/text/number, x/text/message: number formatting order of magnitude error | <!-- Please answer these questions before submitting your issue. Thanks! -->
### What version of Go are you using (`go version`)?
<pre>
$ go version
go version go1.12.1 linux/amd64
</pre>
### Does this issue reproduce with the latest release?
yes
### What operating system and processor architecture are you using (`go env`)?
<details><summary><code>go env</code> Output</summary><br><pre>
$ go env
GOARCH="amd64"
GOBIN=""
GOCACHE="/home/leith/.cache/go-build"
GOEXE=""
GOFLAGS=""
GOHOSTARCH="amd64"
GOHOSTOS="linux"
GOOS="linux"
GOPATH="/home/leith/go"
GOPROXY=""
GORACE=""
GOROOT="/usr/local/go"
GOTMPDIR=""
GOTOOLDIR="/usr/local/go/pkg/tool/linux_amd64"
GCCGO="gccgo"
CC="gcc"
CXX="g++"
CGO_ENABLED="1"
GOMOD=""
CGO_CFLAGS="-g -O2"
CGO_CPPFLAGS=""
CGO_CXXFLAGS="-g -O2"
CGO_FFLAGS="-g -O2"
CGO_LDFLAGS="-g -O2"
PKG_CONFIG="pkg-config"
GOGCCFLAGS="-fPIC -m64 -pthread -fmessage-length=0 -fdebug-prefix-map=/tmp/go-build707188231=/tmp/go-build -gno-record-gcc-switches"
</pre></details>
### What did you do?
<!--
-->
<pre>package main
import (
"fmt"
"golang.org/x/text/message"
"golang.org/x/text/number"
)
func main() {
p := message.NewPrinter(message.MatchLanguage("en"))
n1 := 1.99999
n2 := 0.99999
n3 := 999.9999
fmt.Println(p.Sprintf("%v", number.Decimal(n1, number.Precision(4), number.NoSeparator())))
fmt.Println(p.Sprintf("%v", number.Decimal(n2, number.Precision(4), number.NoSeparator())))
fmt.Println(p.Sprintf("%v", number.Decimal(n3, number.Precision(4), number.NoSeparator())))
}</pre>
### What did you expect to see?
2
1
1000
### What did you see instead?
2
0.1
100
numbers such as 0.99999 are formatted an order of magnitude too small. | NeedsInvestigation | low | Critical |
429,298,205 | pytorch | Allow tracing of models which output `None` | ## π Feature
Allow tracing of models which output tuples with some attributes equal to `None`
## Motivation
In big complex models, the forward pass should be able to output different information, depending on whether the model is being trained or runs in inference (test) mode. In test mode (the one which usually gets traced) some training outputs can be possibly missing. For example, in RL actor-critic methods, during training I want to visualize/plot the output of the critic. However, at test time, I want to be able to perform inference as fast as possible, which requires running the forward pass only of the actor but not of the critic, and return only the actor output.
## Pitch
```python
class ActorCriticModule(torch.nn.Module):
def __init__(self):
self.actor = torch.nn.Sequential(...)
self.critic = torch.nn.Sequential(...)
def forward(self, x, test_mode: bool) -> tuple:
if test_mode:
critic_out = None
else:
critic_out = self.critic(x)
actor_out = self.actor(x)
return actor_out, critic_out
module = ActorCriticModule()
torch.jit.trace(module, (torch.zeros(), True))
```
At the moment, `torch.jit.trace(module, (torch.zeros(), True))` will fail, because `critic_out` will be `None` and tracing will fail.
## Alternatives
Return tuples of different size all the time. It's too scary to image the ugliness of the resulting code, especially with large models
## Additional context
Applicable to any deeplearning scenario.
cc @suo | oncall: jit,feature,triaged | low | Major |
429,355,458 | create-react-app | Revisit dependency pinning | Pinning all of our dependencies causes issues with the preflight check whenever a new version of a nested dependency is released. `babel-jest` is one example of this. When a new version is released and used by another dependency the preflight check fails until we update our version to match.
We should consider unpinning certain dependencies like `babel-jest` and updating the preflight check to work with carrot versions like `^24.7.1`. It currently only works with exact version numbers. | issue: proposal,issue: needs investigation | medium | Major |
429,388,238 | go | x/build/cmd/gopherbot: ensure that frozen issues still receive mentions | I believe this didn't use to be the case, but if a locked (FrozenDueToAge) issue is referenced by a commit or by another issue, it does not show up in the UI. That's fairly annoying, because it's nice to see what follow-up discussion or changes happened when investigating an old issue.
I don't really know what the solution is here. Complain to GitHub? They surely had to do this for anti-abuse reasons.
/cc @golang/osp-team | Builders,NeedsInvestigation | low | Minor |
429,405,762 | go | net/http: `readLoopPeekFailLocked: <nil>` from client when server sends response immediately | <!-- Please answer these questions before submitting your issue. Thanks! -->
### What version of Go are you using (`go version`)?
<pre>
$ go version
go version go1.12.1 linux/amd64
</pre>
### Does this issue reproduce with the latest release?
Yep. 1.14.1
### What operating system and processor architecture are you using (`go env`)?
<details><summary><code>go env</code> Output</summary><br><pre>
$ go env
GOARCH="amd64"
GOBIN=""
GOCACHE="/home/user/.cache/go-build"
GOEXE=""
GOFLAGS=""
GOHOSTARCH="amd64"
GOHOSTOS="linux"
GOOS="linux"
GOPATH="/home/user/go"
GOPROXY=""
GORACE=""
GOROOT="/opt/go"
GOTMPDIR=""
GOTOOLDIR="/opt/go/pkg/tool/linux_amd64"
GCCGO="/usr/bin/gccgo"
CC="gcc"
CXX="g++"
CGO_ENABLED="1"
GOMOD=""
CGO_CFLAGS="-g -O2"
CGO_CPPFLAGS=""
CGO_CXXFLAGS="-g -O2"
CGO_FFLAGS="-g -O2"
CGO_LDFLAGS="-g -O2"
PKG_CONFIG="pkg-config"
GOGCCFLAGS="-fPIC -m64 -pthread -fmessage-length=0 -fdebug-prefix-map=/tmp/go-build705707104=/tmp/go-build -gno-record-gcc-switches"
</pre></details>
### What did you do?
<!--
If possible, provide a recipe for reproducing the error.
A complete runnable program is good.
A link on play.golang.org is best.
-->
100% reproducible on Linux x64, and play.golang.org. The behavior is unpredictable and the code (see below) to reproduce should be executed few times to got the error and the log.
The error is:
```
Get http://127.0.0.1:9101/path: readLoopPeekFailLocked: <nil>
````
The log is:
```
transport.go:1829: Unsolicited response received on idle HTTP channel starting with "HTTP/1.1 502 Bad Gateway\r\n\r\n"; err=<nil>
```
#### What happens?
HTTP server sends response without reading request and without a delay. This approach is good for proxy servers where a client determined by his IP (i.e. using white-list). Thus, we haven't to read request, if the user is not white-listed.
#### Reproducing
(try few times to got error)
[play.golang.org](https://play.golang.org/p/hXm4_UIz33Q)
```go
package main
import (
"fmt"
"log"
"net"
"net/http"
"net/url"
"sync"
"time"
)
func mustParseURL(uri string) *url.URL {
x, err := url.Parse(uri)
if err != nil {
panic(err)
}
return x
}
func main() {
l, err := net.Listen("tcp", "127.0.0.1:9100")
if err != nil {
log.Fatal(err)
}
go func(l net.Listener) {
for {
c, err := l.Accept()
if err != nil {
log.Fatal(err)
}
go func(c net.Conn) {
defer c.Close()
fmt.Fprint(c, "HTTP/1.1 502 Bad Gateway\r\n\r\n")
}(c)
}
}(l)
time.Sleep(100 * time.Millisecond)
var cl http.Client
cl.Transport = &http.Transport{
Proxy: http.ProxyURL(mustParseURL("http://127.0.0.1:9100/")),
MaxConnsPerHost: 100,
}
const N = 100
var wg sync.WaitGroup
wg.Add(N)
for i := 0; i < N; i++ {
go func() {
defer wg.Done()
resp, err := cl.Get("http://127.0.0.1:9101/path")
if err != nil {
log.Print("error:", err)
return
}
if resp.StatusCode != 502 {
log.Println("status:", resp.StatusCode)
}
resp.Body.Close()
}()
}
wg.Wait()
}
```
Also, playing with the N constant I've got this error for N=10, N=100 and N=1000. For N=1000 this error occurs every time. For N=10 rarely. Seems, it depends on goroutines scheduling.
### What did you expect to see?
Got this error every time or never.
### What did you see instead?
It's unpredictable. That makes testing a bit harder.
### Also
Probably related issues #15253, #15446
| help wanted,NeedsInvestigation | medium | Critical |
429,415,928 | TypeScript | Multidimensional readonly arrays | # Search Terms
readonly array multi multidimensional
## Suggestion
The readonly modifier should work with multidimensional arrays.
## Use Cases
I assumed `readonly number[][]` to be multidimensional array which is readonly, but it translates to `ReadonlyArray<number[]>`, this feels like a footgun.
It is currently also not very intuitive which array is readonly: `ReadonlyArray<number>[]` or `ReadonlyArray<number[]>`, without trying it out.
## Examples
I'd expect `readonly number[][]` to be equal to `ReadonlyArray<ReadonlyArray<number>>`
Explicitely "mixed mutable" arrays could be achieved using parens:
```typescript
(readonly number[])[] == ReadonlyArray<number>[]
readonly (number[])[] == ReadonlyArray<number[]>
```
## Checklist
My suggestion meets these guidelines:
* [ ] This wouldn't be a breaking change in existing TypeScript/JavaScript code
* [x] This wouldn't change the runtime behavior of existing JavaScript code
* [x] This could be implemented without emitting different JS based on the types of the expressions
* [x] This isn't a runtime feature (e.g. library functionality, non-ECMAScript syntax with JavaScript output, etc.)
* [x] This feature would agree with the rest of [TypeScript's Design Goals](https://github.com/Microsoft/TypeScript/wiki/TypeScript-Design-Goals).
| Suggestion,In Discussion | low | Major |
429,421,779 | TypeScript | Feature Request: access inherited protected member in subclasses branched from a base class where the protected member is defined in the base class | <!-- π¨ STOP π¨ π¦π§π’π£ π¨ πΊπ»πΆπ· π¨
Half of all issues filed here are duplicates, answered in the FAQ, or not appropriate for the bug tracker. Please read the FAQ first, especially the "Common Feature Requests" section.
-->
## Search Terms
- typescript protected class branch
- typescript access protected in sibling
## Suggestion
Allow subclasses to access inherited protected members across instances of the subclasses.
## Use Cases
There are use cases when we'd like subclass branches that inherit a protected member to be able to access the inherited protected member across instances of the branched subclasses.
There are also use cases where subclasses cause invariants by narrowing the type of an inherited member, which we should be able to guard against at compile time.
## Examples
This [PlayGround example](http://www.typescriptlang.org/play/#src=let%20counter%20%3D%200%0D%0A%0D%0Aclass%20Base%20%7B%20%0D%0A%20%20%20%20protected%20foo%20%3D%20%2B%2Bcounter%0D%0A%7D%0D%0A%0D%0Aclass%20A%20extends%20Base%20%7B%0D%0A%20%20%20%20getOtherFoo(other%3A%20Base)%20%7B%0D%0A%20%20%20%20%20%20%20%20return%20other.foo%0D%0A%20%20%20%20%7D%0D%0A%7D%0D%0A%0D%0Aclass%20B%20extends%20Base%20%7B%0D%0A%7D%0D%0A%0D%0Aconst%20a%20%3D%20new%20A%0D%0Aconst%20b%20%3D%20new%20B%0D%0A%0D%0Aconsole.log(a.getOtherFoo(b))%20%2F%2F%202%0D%0A%0D%0Aclass%20MyClass%20%7B%0D%0A%20%20%20%20protected%20protectedProperty%3A%20number%20%3D%201%0D%0A%7D%0D%0A%0D%0Aclass%20MySubClass%20extends%20MyClass%20%7B%0D%0A%20%20%20%20protected%20protectedProperty%3A%20number%20%3D%202%0D%0A%20%20%20%20static%20compare(a%3A%20MyClass%2C%20b%3A%20MySubClass)%3A%20number%20%7B%0D%0A%20%20%20%20%20%20%20%20return%20a.protectedProperty%20-%20b.protectedProperty%3B%0D%0A%20%20%20%20%7D%0D%0A%7D%0D%0A%0D%0Aconst%20m1%20%3D%20new%20MyClass%0D%0Aconst%20m2%20%3D%20new%20MySubClass%0D%0A%0D%0Aconsole.log(MySubClass.compare(m1%2C%20m2))%20%2F%2F%20-1) doesn't work, to illustrate the current limitation.
I have a use case, where I have a `TreeNode` sort of class with protected properties, then I have subclasses of the TreeNode, one called `Mesh`, and the other called `Scene`, where the Scene needs to traverse the tree to run logic using the protected members (thus reading the protected member of all instances of `Mesh`), but TypeScript won't allow it.
The code that is `Scene`-specific lives inside the `Scene` class (because that makes sense).
To make it work, I'd have to move that code into the common base class `TreeNode`, but then it will be messy and un-organized.
FWIW, I have the same problem in my runtime JS class implementation, [lowclass](https://github.com/trusktr/lowclass), which I'm now thinking about solving with an option.
In general, I think that well-designed classes will benefit from this feature, and that poor coding practices will lead to issues with this (which is why the compiler should catch the problems in those cases). (f.e. if base class specifies a protected member of type `number | string`, and a sibling subclass changes it to `number`, then the compiler can obviously catch a problem when another sibling subclass wants to read from the other one and hasn't narrowed the type to `number` as well.)
## implementation ideas
A subset of the following ideas might do the trick:
- a compiler option to allow or disallow this feature
- We can detect invariants at compile time. From [this post](https://stackoverflow.com/questions/1904782/whats-the-real-reason-for-preventing-protected-member-access-through-a-base-sib),
> Other sibling classes might add invariants that your class doesn't know about
In the above example, if the types agree in the subclasses, we can let it work, and throw a runtime error only when there is a type mismatch. But suppose in my above example that `Scene` and `Mesh` don't modify the inherited protected type, and only read it or set it. The compiler can easily ensure that things are okay.
- disallow subclasses from modifying types of inherited members
- perhaps a compiler option for this
- this can prevent subclasses of subclasses of subclasses from being so far removed (not resembling their base class at all), by forcing subclasses to add new features on new properties instead of existing ones
- Follow the same rules as public, which is not guarded against this. An invariant problem can happen across siblings inheriting and modifying the member from the base class, yet the compiler will not stop it.
- another compiler option
Mainly, my reasoning above is that there is a way to make this work with some semantics and/or options, and it is okay if those are specific to TypeScript. We won't invent new wheels, but we can improve existing wheels instead of copying them.
## Checklist
My suggestion meets these guidelines:
* [ ] This wouldn't be a breaking change in existing TypeScript/JavaScript code _**not sure**_
* [x] This wouldn't change the runtime behavior of existing JavaScript code
* [x] This could be implemented without emitting different JS based on the types of the expressions
* [x] This isn't a runtime feature (e.g. library functionality, non-ECMAScript syntax with JavaScript output, etc.)
* [x] This feature would agree with the rest of [TypeScript's Design Goals](https://github.com/Microsoft/TypeScript/wiki/TypeScript-Design-Goals).
**Related Issues:**
- https://github.com/Microsoft/TypeScript/issues/1
- https://github.com/Microsoft/TypeScript/issues/8512
- https://github.com/Microsoft/TypeScript/issues/10637
- https://github.com/Microsoft/TypeScript/issues/11278
- https://github.com/Microsoft/TypeScript/issues/11832
- https://github.com/Microsoft/TypeScript/issues/22900
| Suggestion,Awaiting More Feedback | low | Critical |
429,431,436 | rust | Undefined symbols for architecture x86_64 | First of all I apologise for the long steps needed to reproduce this issue.
### Error
```
= note: Undefined symbols for architecture x86_64:
"_$LT$openm44..orientation..FLAT$u20$as$u20$core..ops..deref..Deref$GT$::deref::__stability::LAZY::ha1a1ee59629f5d0d (.llvm.14922800789097485937)", referenced from:
openm44::game::Game::new::he00edae092ca94fd in libopenm44-b18ac1b6021fb1a6.rlib(openm44-b18ac1b6021fb1a6.34ixt68ftx3uvjkh.rcgu.o)
ld: symbol(s) not found for architecture x86_64
clang: error: linker command failed with exit code 1 (use -v to see invocation)
```
## Steps to reproduce
This error is quite spurious, it seems to be fixed by changing the `opt-level` back to `0`, if you change the code first it could not error or it could hang forever. It doesn't seem to be related to incremental. I've also had this error happen when using `HashMap::get_mut` but I couldn't reproduce that case.
* Clone XAMPPRocky/openm44
* Checkout the `linker_error` branch.
* Run `cargo run`. (I'm sorry, this has 160β200 dependencies)
* You should get the below output
```
thread 'main' panicked at 'called `Result::unwrap()` on an `Err` value: IOError(Os { code: 2, kind: NotFound, message: "No such file or directory" })', src/libcore/result.rs:997:5
note: Run with `RUST_BACKTRACE=1` environment variable to display a backtrace.
```
* Change `&*crate::orientation::POINTY` on [line 62 of `src/layout`][code-line] to `&*crate::orientation::FLAT`
* Run `cargo run`
## Rustc
```
rustc 1.33.0 (2aa4c46cf 2019-02-28)
binary: rustc
commit-hash: 2aa4c46cfdd726e97360c2734835aa3515e8c858
commit-date: 2019-02-28
host: x86_64-apple-darwin
release: 1.33.0
LLVM version: 8.0
```
## Full output
```
Fresh ucd-util v0.1.3
Fresh glob v0.2.11
Fresh lazy_static v1.3.0
Fresh cfg-if v0.1.7
Fresh utf8-ranges v1.0.2
Fresh unicode-xid v0.1.0
Fresh unicode-width v0.1.5
Fresh ansi_term v0.11.0
Fresh vec_map v0.8.1
Fresh strsim v0.7.0
Fresh bitflags v1.0.4
Fresh peeking_take_while v0.1.2
Fresh autocfg v0.1.2
Fresh cc v1.0.34
Fresh rand_core v0.4.0
Fresh nodrop v0.1.13
Fresh void v1.0.2
Fresh unicode-xid v0.0.4
Fresh adler32 v1.0.3
Fresh linked-hash-map v0.5.2
Fresh rustc-demangle v0.1.13
Fresh rawpointer v0.1.0
Fresh fnv v1.0.6
Fresh bitflags v0.7.0
Fresh color_quant v1.0.1
Fresh quote v0.3.15
Fresh lzw v0.10.0
Fresh approx v0.1.1
Fresh lazy_static v0.2.11
Fresh smallvec v0.6.9
Fresh xi-unicode v0.1.0
Fresh claxon v0.3.2
Fresh podio v0.1.6
Fresh dtoa v0.4.3
Fresh hound v3.4.0
Fresh either v1.5.2
Fresh app_dirs2 v2.0.3
Fresh regex-syntax v0.5.6
Fresh thread_local v0.3.6
Fresh log v0.4.6
Fresh proc-macro2 v0.2.3
Fresh textwrap v0.10.0
Fresh xml-rs v0.7.0
Fresh draw_state v0.8.0
Fresh rand_core v0.3.1
Fresh unreachable v1.0.0
Fresh synom v0.11.3
Fresh inflate v0.4.5
Fresh yaml-rust v0.4.3
Fresh gif v0.10.1
Fresh unicode-normalization v0.1.8
Fresh itertools v0.7.11
Fresh memchr v2.2.0
Fresh libc v0.2.51
Fresh libloading v0.4.3
Fresh log v0.3.9
Fresh quote v0.4.2
Fresh num-traits v0.2.6
Fresh proc-macro2 v0.4.27
Fresh khronos_api v2.2.0
Fresh arrayvec v0.4.10
Fresh rand_isaac v0.1.1
Fresh rand_hc v0.1.0
Fresh rand_xorshift v0.1.1
Fresh byteorder v1.3.1
Fresh typenum v1.10.0
Fresh syn v0.11.11
Fresh matrixmultiply v0.1.15
Fresh serde v1.0.90
Fresh aho-corasick v0.6.10
Fresh memchr v1.0.2
Fresh atty v0.2.11
Fresh which v1.0.5
Fresh rand_jitter v0.1.3
Fresh rand v0.4.6
Fresh rand_os v0.1.3
Fresh time v0.1.42
Fresh core-foundation-sys v0.5.1
Fresh clang-sys v0.21.2
Fresh sdl2-sys v0.31.0
Fresh num-traits v0.1.43
Fresh num-integer v0.1.39
Fresh num-complex v0.1.43
Fresh quote v0.6.11
Fresh gl_generator v0.9.0
Fresh rand_pcg v0.1.2
Fresh rand_chacha v0.1.1
Fresh backtrace v0.3.14
Fresh bzip2-sys v0.1.7
Fresh deflate v0.7.19
Fresh stb_truetype v0.2.6
Fresh ogg v0.4.1
Fresh jpeg-decoder v0.1.15
Fresh generic-array v0.8.3
Fresh smart-default v0.2.0
Fresh toml v0.4.10
Fresh serde_yaml v0.8.8
Fresh regex v0.2.11
Fresh nom v3.2.1
Fresh clap v2.32.0
Fresh rand v0.3.23
Fresh msdos_time v0.1.6
Fresh euclid v0.17.3
Fresh sid v0.5.2
Fresh ordered-float v0.5.2
Fresh num-iter v0.1.37
Fresh num-rational v0.1.42
Fresh alga v0.5.4
Fresh syn v0.15.30
Fresh rand v0.6.5
Fresh error-chain v0.10.0
Fresh bzip2 v0.3.3
Fresh lewton v0.5.2
Fresh env_logger v0.4.3
Fresh cexpr v0.2.3
Fresh cgmath v0.14.1
Fresh lyon_geom v0.10.1
Fresh rusttype v0.5.2
Fresh num v0.1.42
Fresh png v0.12.0
Fresh nalgebra v0.14.4
Fresh derivative v1.0.2
Fresh num-derive v0.2.4
Fresh serde_derive v1.0.90
Fresh twox-hash v1.1.2
Fresh yaml-merge-keys v0.3.0
Fresh zip v0.3.3
Fresh bindgen v0.32.3
Fresh lyon_path v0.10.1
Fresh sdl2 v0.31.0
Fresh gfx_core v0.8.3
Fresh image v0.19.0
Fresh gfx_gl v0.5.0
Fresh lyon_tessellation v0.10.2
Fresh gfx v0.17.1
Fresh gfx_device_gl v0.15.5
Fresh lyon v0.10.2
Fresh gfx_glyph v0.10.2
Fresh gfx_window_sdl v0.8.0
Fresh coreaudio-sys v0.2.2
Fresh coreaudio-rs v0.9.1
Fresh cpal v0.8.2
Fresh rodio v0.7.0
Fresh ggez v0.4.4
Compiling openm44 v0.1.0 (/Users/aep/src/openm44)
Running `rustc --crate-name openm44 src/main.rs --color always --crate-type bin --emit=dep-info,link -C opt-level=2 -C debuginfo=2 -C debug-assertions=on -C metadata=4cad5c9c2117641f -C extra-filename=-4cad5c9c2117641f --out-dir /Users/aep/src/openm44/target/debug/deps -C incremental=/Users/aep/src/openm44/target/debug/incremental -L dependency=/Users/aep/src/openm44/target/debug/deps --extern ggez=/Users/aep/src/openm44/target/debug/deps/libggez-a1434b343f73fabd.rlib --extern lazy_static=/Users/aep/src/openm44/target/debug/deps/liblazy_static-33c51f17c72ef9f4.rlib --extern openm44=/Users/aep/src/openm44/target/debug/deps/libopenm44-b18ac1b6021fb1a6.rlib --extern rand=/Users/aep/src/openm44/target/debug/deps/librand-f40a137293de3ea6.rlib --extern serde=/Users/aep/src/openm44/target/debug/deps/libserde-ca9fc1cb9204a3a0.rlib --extern serde_derive=/Users/aep/src/openm44/target/debug/deps/libserde_derive-54b69c06e37e5ac8.dylib --extern serde_yaml=/Users/aep/src/openm44/target/debug/deps/libserde_yaml-266327b739d45545.rlib --extern yaml_merge_keys=/Users/aep/src/openm44/target/debug/deps/libyaml_merge_keys-f9f4e811e1c60aab.rlib -C target-cpu=native -L native=/Users/aep/src/openm44/target/debug/build/bzip2-sys-62ba1ecf6f24750e/out/lib`
error: linking with `cc` failed: exit code: 1
|
= note: "cc" "-m64" "-L" "/Users/aep/.rustup/toolchains/stable-x86_64-apple-darwin/lib/rustlib/x86_64-apple-darwin/lib" "/Users/aep/src/openm44/target/debug/deps/openm44-4cad5c9c2117641f.12i7qeo4r6iva8bf.rcgu.o" "/Users/aep/src/openm44/target/debug/deps/openm44-4cad5c9c2117641f.181nvzg7z39z93cx.rcgu.o" "/Users/aep/src/openm44/target/debug/deps/openm44-4cad5c9c2117641f.1ln5a3o626etidrj.rcgu.o" "/Users/aep/src/openm44/target/debug/deps/openm44-4cad5c9c2117641f.1u2zi85kxcfop7v5.rcgu.o" "/Users/aep/src/openm44/target/debug/deps/openm44-4cad5c9c2117641f.24fj0kwpq7wly2kq.rcgu.o" "/Users/aep/src/openm44/target/debug/deps/openm44-4cad5c9c2117641f.26al8s29656fi14s.rcgu.o" "/Users/aep/src/openm44/target/debug/deps/openm44-4cad5c9c2117641f.274jjzkvtwxfohx6.rcgu.o" "/Users/aep/src/openm44/target/debug/deps/openm44-4cad5c9c2117641f.2a6nwire5c2gfh5l.rcgu.o" "/Users/aep/src/openm44/target/debug/deps/openm44-4cad5c9c2117641f.2etjnmp60cja3ipy.rcgu.o" "/Users/aep/src/openm44/target/debug/deps/openm44-4cad5c9c2117641f.2exg2x5ig2lz1u1e.rcgu.o" "/Users/aep/src/openm44/target/debug/deps/openm44-4cad5c9c2117641f.2onu3k2v584e1rsr.rcgu.o" "/Users/aep/src/openm44/target/debug/deps/openm44-4cad5c9c2117641f.2qqtfxpaoyevh5i0.rcgu.o" "/Users/aep/src/openm44/target/debug/deps/openm44-4cad5c9c2117641f.2sksa8cm7bmt8qz3.rcgu.o" "/Users/aep/src/openm44/target/debug/deps/openm44-4cad5c9c2117641f.38vd7f4nldc7e2pw.rcgu.o" "/Users/aep/src/openm44/target/debug/deps/openm44-4cad5c9c2117641f.3b0356h3b1uknzj8.rcgu.o" "/Users/aep/src/openm44/target/debug/deps/openm44-4cad5c9c2117641f.3caxon3dyy6ctm3m.rcgu.o" "/Users/aep/src/openm44/target/debug/deps/openm44-4cad5c9c2117641f.3elsj62444z9ap3i.rcgu.o" "/Users/aep/src/openm44/target/debug/deps/openm44-4cad5c9c2117641f.3rt28by0folzosgx.rcgu.o" "/Users/aep/src/openm44/target/debug/deps/openm44-4cad5c9c2117641f.42zdkclvkc44lzh6.rcgu.o" "/Users/aep/src/openm44/target/debug/deps/openm44-4cad5c9c2117641f.49a10iryjb9ecve6.rcgu.o" "/Users/aep/src/openm44/target/debug/deps/openm44-4cad5c9c2117641f.4bild5kndfyixjqo.rcgu.o" "/Users/aep/src/openm44/target/debug/deps/openm44-4cad5c9c2117641f.4hr1vmqov7wp8bmp.rcgu.o" "/Users/aep/src/openm44/target/debug/deps/openm44-4cad5c9c2117641f.52qqzlro2gfkdnii.rcgu.o" "/Users/aep/src/openm44/target/debug/deps/openm44-4cad5c9c2117641f.54ttn0c7jc93b1a3.rcgu.o" "/Users/aep/src/openm44/target/debug/deps/openm44-4cad5c9c2117641f.djappinvxq645yx.rcgu.o" "/Users/aep/src/openm44/target/debug/deps/openm44-4cad5c9c2117641f.gtfzwk2989i25jy.rcgu.o" "/Users/aep/src/openm44/target/debug/deps/openm44-4cad5c9c2117641f.wkfxrfbson20odh.rcgu.o" "-o" "/Users/aep/src/openm44/target/debug/deps/openm44-4cad5c9c2117641f" "/Users/aep/src/openm44/target/debug/deps/openm44-4cad5c9c2117641f.4ttsxlkzn8w9id8u.rcgu.o" "-Wl,-dead_strip" "-nodefaultlibs" "-L" "/Users/aep/src/openm44/target/debug/deps" "-L" "/Users/aep/src/openm44/target/debug/build/bzip2-sys-62ba1ecf6f24750e/out/lib" "-L" "/Users/aep/.rustup/toolchains/stable-x86_64-apple-darwin/lib/rustlib/x86_64-apple-darwin/lib" "/Users/aep/src/openm44/target/debug/deps/libopenm44-b18ac1b6021fb1a6.rlib" "/Users/aep/src/openm44/target/debug/deps/libyaml_merge_keys-f9f4e811e1c60aab.rlib" "/Users/aep/src/openm44/target/debug/deps/libitertools-da42954271f41c43.rlib" "/Users/aep/src/openm44/target/debug/deps/libeither-a8dca019ee877371.rlib" "/Users/aep/src/openm44/target/debug/deps/liberror_chain-b4ef00902d1630f0.rlib" "/Users/aep/src/openm44/target/debug/deps/libserde_yaml-266327b739d45545.rlib" "/Users/aep/src/openm44/target/debug/deps/libyaml_rust-0440f1db9fcdc51a.rlib" "/Users/aep/src/openm44/target/debug/deps/libdtoa-f0a0ed83f6be8de3.rlib" "/Users/aep/src/openm44/target/debug/deps/libggez-a1434b343f73fabd.rlib" "/Users/aep/src/openm44/target/debug/deps/libzip-9768b289f4f54a06.rlib" "/Users/aep/src/openm44/target/debug/deps/libpodio-ebf8660ca9843743.rlib" "/Users/aep/src/openm44/target/debug/deps/libmsdos_time-4f8af4bee8357d82.rlib" "/Users/aep/src/openm44/target/debug/deps/libtime-c743629c49551248.rlib" "/Users/aep/src/openm44/target/debug/deps/libbzip2-879503cca28d5114.rlib" "/Users/aep/src/openm44/target/debug/deps/libbzip2_sys-685000300fdef309.rlib" "/Users/aep/src/openm44/target/debug/deps/libtoml-c422bd572fb04710.rlib" "/Users/aep/src/openm44/target/debug/deps/libserde-ca9fc1cb9204a3a0.rlib" "/Users/aep/src/openm44/target/debug/deps/librodio-4001d1c7890311b6.rlib" "/Users/aep/src/openm44/target/debug/deps/libcgmath-58c5d98694f1398d.rlib" "/Users/aep/src/openm44/target/debug/deps/liblewton-1dfd2d832b6881df.rlib" "/Users/aep/src/openm44/target/debug/deps/libogg-e53b483d3548f9cf.rlib" "/Users/aep/src/openm44/target/debug/deps/libhound-f07258d9b948a939.rlib" "/Users/aep/src/openm44/target/debug/deps/libcpal-2f77b124e89d58b0.rlib" "/Users/aep/src/openm44/target/debug/deps/libcore_foundation_sys-a3db1d934c1809ed.rlib" "/Users/aep/src/openm44/target/debug/deps/libcoreaudio-46d5671dfe58db3d.rlib" "/Users/aep/src/openm44/target/debug/deps/libcoreaudio_sys-5b6f08e874b0b8ed.rlib" "/Users/aep/src/openm44/target/debug/deps/libclaxon-ed599c61d155f564.rlib" "/Users/aep/src/openm44/target/debug/deps/libnalgebra-a983ac4228555f0c.rlib" "/Users/aep/src/openm44/target/debug/deps/libalga-3e6dccf93c474b5c.rlib" "/Users/aep/src/openm44/target/debug/deps/libnum_complex-ad95609806e9688b.rlib" "/Users/aep/src/openm44/target/debug/deps/libmatrixmultiply-29003071f5246473.rlib" "/Users/aep/src/openm44/target/debug/deps/librawpointer-67ec6ede1e3269d4.rlib" "/Users/aep/src/openm44/target/debug/deps/libgeneric_array-f5d9cd295cb9bc5e.rlib" "/Users/aep/src/openm44/target/debug/deps/libtypenum-8201d7b95e516bfe.rlib" "/Users/aep/src/openm44/target/debug/deps/liblyon-8c618434f0b75bd8.rlib" "/Users/aep/src/openm44/target/debug/deps/liblyon_tessellation-084100f4b29a0642.rlib" "/Users/aep/src/openm44/target/debug/deps/libsid-f6fa70e3bb710eef.rlib" "/Users/aep/src/openm44/target/debug/deps/liblyon_path-8b824a60b1dbe3ee.rlib" "/Users/aep/src/openm44/target/debug/deps/liblyon_geom-aaf9e8a744f4d8c6.rlib" "/Users/aep/src/openm44/target/debug/deps/libeuclid-37b0315f55794618.rlib" "/Users/aep/src/openm44/target/debug/deps/libimage-a5573001f432c109.rlib" "/Users/aep/src/openm44/target/debug/deps/libgif-0febc02fae3959b0.rlib" "/Users/aep/src/openm44/target/debug/deps/libcolor_quant-d76ac73ef978df1a.rlib" "/Users/aep/src/openm44/target/debug/deps/libjpeg_decoder-19ab8c3344166d40.rlib" "/Users/aep/src/openm44/target/debug/deps/libpng-57c7f391c8897fbb.rlib" "/Users/aep/src/openm44/target/debug/deps/libdeflate-ee3dfcbff8232c1a.rlib" "/Users/aep/src/openm44/target/debug/deps/libinflate-6eedb925c73f3278.rlib" "/Users/aep/src/openm44/target/debug/deps/libadler32-9e6af50984ed49e8.rlib" "/Users/aep/src/openm44/target/debug/deps/liblzw-01303f353d1c7f13.rlib" "/Users/aep/src/openm44/target/debug/deps/libnum_rational-dd6671692fd920ab.rlib" "/Users/aep/src/openm44/target/debug/deps/libgfx_window_sdl-e9e2193884335211.rlib" "/Users/aep/src/openm44/target/debug/deps/libsdl2-0b1fb598a985c1e0.rlib" "/Users/aep/src/openm44/target/debug/deps/librand-f46aa1ebbb41f34f.rlib" "/Users/aep/src/openm44/target/debug/deps/librand-89a73b97a78aa358.rlib" "/Users/aep/src/openm44/target/debug/deps/libsdl2_sys-3f3acb67c2e7e9a1.rlib" "/Users/aep/src/openm44/target/debug/deps/libbitflags-bea0fd9dd4290bd9.rlib" "/Users/aep/src/openm44/target/debug/deps/liblazy_static-38decb78e5e72f5f.rlib" "/Users/aep/src/openm44/target/debug/deps/libnum-9a059b9a4ac911a6.rlib" "/Users/aep/src/openm44/target/debug/deps/libnum_iter-8dd5e89344d0a5e9.rlib" "/Users/aep/src/openm44/target/debug/deps/libnum_integer-77b5addd0c00616e.rlib" "/Users/aep/src/openm44/target/debug/deps/libgfx_glyph-005afb89fa1f9618.rlib" "/Users/aep/src/openm44/target/debug/deps/libxi_unicode-afa9d41d5574c42e.rlib" "/Users/aep/src/openm44/target/debug/deps/libunicode_normalization-694d13e21cea054a.rlib" "/Users/aep/src/openm44/target/debug/deps/libsmallvec-cd5fa54f93171c59.rlib" "/Users/aep/src/openm44/target/debug/deps/libtwox_hash-ba62ed23f1519a44.rlib" "/Users/aep/src/openm44/target/debug/deps/librand-f40a137293de3ea6.rlib" "/Users/aep/src/openm44/target/debug/deps/librand_xorshift-e3d2d7e4b4bbefbf.rlib" "/Users/aep/src/openm44/target/debug/deps/librand_pcg-16b56105c2c07cfe.rlib" "/Users/aep/src/openm44/target/debug/deps/librand_hc-b8724c65a1b7398d.rlib" "/Users/aep/src/openm44/target/debug/deps/librand_chacha-5d588c732442d516.rlib" "/Users/aep/src/openm44/target/debug/deps/librand_isaac-1792dc6a6ffd49cb.rlib" "/Users/aep/src/openm44/target/debug/deps/librand_core-01e531db9f4b36ef.rlib" "/Users/aep/src/openm44/target/debug/deps/librand_os-3ff6cc24ea10f0b0.rlib" "/Users/aep/src/openm44/target/debug/deps/librand_jitter-d56f4a994389ee93.rlib" "/Users/aep/src/openm44/target/debug/deps/librand_core-7f4cf1d9844170c0.rlib" "/Users/aep/src/openm44/target/debug/deps/librusttype-aa9764df7618e90a.rlib" "/Users/aep/src/openm44/target/debug/deps/liblinked_hash_map-408e23c21b7deb43.rlib" "/Users/aep/src/openm44/target/debug/deps/libfnv-ef89a5a11e4346ed.rlib" "/Users/aep/src/openm44/target/debug/deps/libstb_truetype-62a8130afb1f9dd8.rlib" "/Users/aep/src/openm44/target/debug/deps/libbyteorder-c33973dc5fb31ddb.rlib" "/Users/aep/src/openm44/target/debug/deps/libarrayvec-7fc74ea4fb17974c.rlib" "/Users/aep/src/openm44/target/debug/deps/libnodrop-7d4a05ccab346fb1.rlib" "/Users/aep/src/openm44/target/debug/deps/libapprox-7e51d710922e2894.rlib" "/Users/aep/src/openm44/target/debug/deps/libordered_float-8ba5ee635a4d7e35.rlib" "/Users/aep/src/openm44/target/debug/deps/libunreachable-889eebb14e3cbc3f.rlib" "/Users/aep/src/openm44/target/debug/deps/libvoid-2b9c3bf882a6c13f.rlib" "/Users/aep/src/openm44/target/debug/deps/libnum_traits-2dc513570ab3b1e1.rlib" "/Users/aep/src/openm44/target/debug/deps/libnum_traits-c6230151cf74133e.rlib" "/Users/aep/src/openm44/target/debug/deps/libbacktrace-34ea63b9a3c9addc.rlib" "/Users/aep/src/openm44/target/debug/deps/librustc_demangle-7d58dd655468a5ab.rlib" "/Users/aep/src/openm44/target/debug/deps/liblibc-20614a28e625a397.rlib" "/Users/aep/src/openm44/target/debug/deps/libgfx_device_gl-d5010af77c85972d.rlib" "/Users/aep/src/openm44/target/debug/deps/libgfx_gl-bf2f7114d947ada8.rlib" "/Users/aep/src/openm44/target/debug/deps/libgfx-03a6071d14f71753.rlib" "/Users/aep/src/openm44/target/debug/deps/libgfx_core-421bb4f17f9947ec.rlib" "/Users/aep/src/openm44/target/debug/deps/libdraw_state-b2249afff6ffb860.rlib" "/Users/aep/src/openm44/target/debug/deps/libbitflags-46ff434c2a502b54.rlib" "/Users/aep/src/openm44/target/debug/deps/liblog-8d68c4f87b72cd0f.rlib" "/Users/aep/src/openm44/target/debug/deps/libcfg_if-4c92674b38dfed8b.rlib" "/Users/aep/src/openm44/target/debug/deps/libapp_dirs2-2347f827488b9c67.rlib" "/Users/aep/src/openm44/target/debug/deps/liblazy_static-33c51f17c72ef9f4.rlib" "/Users/aep/.rustup/toolchains/stable-x86_64-apple-darwin/lib/rustlib/x86_64-apple-darwin/lib/libstd-d4fbe66ddea5f3ce.rlib" "/Users/aep/.rustup/toolchains/stable-x86_64-apple-darwin/lib/rustlib/x86_64-apple-darwin/lib/libpanic_unwind-cb02f3c2aa14313b.rlib" "/Users/aep/.rustup/toolchains/stable-x86_64-apple-darwin/lib/rustlib/x86_64-apple-darwin/lib/libbacktrace_sys-b0895307a49d7b98.rlib" "/Users/aep/.rustup/toolchains/stable-x86_64-apple-darwin/lib/rustlib/x86_64-apple-darwin/lib/libunwind-5751ec7e6e80fbea.rlib" "/Users/aep/.rustup/toolchains/stable-x86_64-apple-darwin/lib/rustlib/x86_64-apple-darwin/lib/librustc_demangle-136c67c44acb8989.rlib" "/Users/aep/.rustup/toolchains/stable-x86_64-apple-darwin/lib/rustlib/x86_64-apple-darwin/lib/liblibc-440e2779940c5e25.rlib" "/Users/aep/.rustup/toolchains/stable-x86_64-apple-darwin/lib/rustlib/x86_64-apple-darwin/lib/liballoc-089d48ff1bba5f70.rlib" "/Users/aep/.rustup/toolchains/stable-x86_64-apple-darwin/lib/rustlib/x86_64-apple-darwin/lib/librustc_std_workspace_core-9d6280dd79b180e2.rlib" "/Users/aep/.rustup/toolchains/stable-x86_64-apple-darwin/lib/rustlib/x86_64-apple-darwin/lib/libcore-f948d62f596dcd3d.rlib" "/Users/aep/.rustup/toolchains/stable-x86_64-apple-darwin/lib/rustlib/x86_64-apple-darwin/lib/libcompiler_builtins-35232c4af1148867.rlib" "-framework" "CoreFoundation" "-framework" "AudioUnit" "-framework" "CoreAudio" "-lSDL2" "-framework" "Security" "-lSystem" "-lresolv" "-lc" "-lm"
= note: Undefined symbols for architecture x86_64:
"_$LT$openm44..orientation..FLAT$u20$as$u20$core..ops..deref..Deref$GT$::deref::__stability::LAZY::ha1a1ee59629f5d0d (.llvm.14922800789097485937)", referenced from:
openm44::game::Game::new::he00edae092ca94fd in libopenm44-b18ac1b6021fb1a6.rlib(openm44-b18ac1b6021fb1a6.34ixt68ftx3uvjkh.rcgu.o)
ld: symbol(s) not found for architecture x86_64
clang: error: linker command failed with exit code 1 (use -v to see invocation)
error: aborting due to previous error
error: Could not compile `openm44`.
Caused by:
process didn't exit successfully: `rustc --crate-name openm44 src/main.rs --color always --crate-type bin --emit=dep-info,link -C opt-level=2 -C debuginfo=2 -C debug-assertions=on -C metadata=4cad5c9c2117641f -C extra-filename=-4cad5c9c2117641f --out-dir /Users/aep/src/openm44/target/debug/deps -C incremental=/Users/aep/src/openm44/target/debug/incremental -L dependency=/Users/aep/src/openm44/target/debug/deps --extern ggez=/Users/aep/src/openm44/target/debug/deps/libggez-a1434b343f73fabd.rlib --extern lazy_static=/Users/aep/src/openm44/target/debug/deps/liblazy_static-33c51f17c72ef9f4.rlib --extern openm44=/Users/aep/src/openm44/target/debug/deps/libopenm44-b18ac1b6021fb1a6.rlib --extern rand=/Users/aep/src/openm44/target/debug/deps/librand-f40a137293de3ea6.rlib --extern serde=/Users/aep/src/openm44/target/debug/deps/libserde-ca9fc1cb9204a3a0.rlib --extern serde_derive=/Users/aep/src/openm44/target/debug/deps/libserde_derive-54b69c06e37e5ac8.dylib --extern serde_yaml=/Users/aep/src/openm44/target/debug/deps/libserde_yaml-266327b739d45545.rlib --extern yaml_merge_keys=/Users/aep/src/openm44/target/debug/deps/libyaml_merge_keys-f9f4e811e1c60aab.rlib -C target-cpu=native -L native=/Users/aep/src/openm44/target/debug/build/bzip2-sys-62ba1ecf6f24750e/out/lib` (exit code: 1)
```
[code-line]: https://github.com/XAMPPRocky/openm44/blob/bbd558649672a5dbb359f4257205c19ea49c5726/src/layout.rs#L62 | A-linkage,T-compiler,C-bug,E-needs-mcve | low | Critical |
429,443,712 | go | x/build/cmd/coordinator: bound the number of times coordinator can reschedule a failed build due to buildlet disappearing | The plan9-386 builders are dying halfway through (buildlet crashing?) and restarting the build forever.
We should bound the number of retries in the coordinator so plan9's flakiness doesn't waste our resources forever.
/cc @0intro @dmitshur
| OS-Plan9,Builders,NeedsFix | low | Critical |
429,467,263 | go | cmd/compile: intrinsify math/bits.RotateLeft{32,64} on all architectures | https://github.com/golang/go/issues/21536#issuecomment-480003894 reminded me about this. Currently the intrinsic is only implemented on amd64, arm64, ppc64 and s390x. We should enable it everywhere. It will need tests in `tests/codegen`. Could be a good beginner compiler issue to tackle.
CLs so far:
* amd64: https://go-review.googlesource.com/c/go/+/132435
* arm64: https://go-review.googlesource.com/c/go/+/122542
* ppc64: https://go-review.googlesource.com/c/go/+/163760
* s390x: https://go-review.googlesource.com/c/go/+/133035 | Performance,help wanted,NeedsFix,compiler/runtime | low | Major |
429,474,794 | rust | Improved error message when forgetting to specify default type parameter | It's kind of hard to put this scenario into words, so let me just show you a short example of what I mean:
```
struct Foo<T=u32>(T);
impl<T> Foo<T> {
fn get(self) -> T { self.0 }
}
fn bar<T>(foo: Foo) {
let value: T = foo.get();
}
```
gives the following error:
```
error[E0308]: mismatched types
--> src/main.rs:9:20
|
9 | let value: T = foo.get();
| ^^^^^^^^^ expected type parameter, found u32
|
= note: expected type `T`
found type `u32`
```
This just came up on the nom gitter (in a more complex code sample) and was somewhat confusing to debug. Maybe the error message could hint about adding the generic type parameter to the argument type? I.e. suggest something along the lines of "did you mean Foo<T>" or even "... instead of Foo<T=u32>". Currently u32 just seems to appear out of nowhere.
I am, however, not quite sure how easy this would be to improve or even detect. | C-enhancement,A-diagnostics,T-compiler | low | Critical |
429,517,970 | pytorch | [FR] torch.dist along a dimension | triaged,function request,module: reductions | low | Minor |
|
429,528,637 | pytorch | Provide option to use alias method in Categorical.sample() | Ref to https://github.com/pytorch/pytorch/issues/4115. The alias method provides a very efficient way to sample from a categorical distribution in cases where the underlying distribution is static with many categories, and we want to sample from it many times. Once the alias table is built, sampling takes constant time w.r.t. the number of categories.
@vishwakftw has exposed the bindings to the sampler via `torch._multinomial_alias_setup` and `torch._multinomial_alias_draw` functions. We should make this available in `torch.distributions.categorical`. Since the `torch._multinomial_alias_setup` function is restricted to 1-D probability table, we cannot use this sampler with batched (and, distinct) `probs` argument.
My proposal would be to have the `Categorical.__init__` method take in an optional keyword argument `use_alias=False`. If the `probs/logits` have the correct shape, then we can construct and store the alias table. If `probs` is batched, we can raise a warning, set `use_alias=False` and use the default sampler. We will also need to make sure that `expand` works fine even if the `probs` tensor is subsequently expanded.
cc. @vishwakftw, @fmassa, @HobbitLong | module: distributions,triaged | low | Minor |
429,548,024 | TypeScript | Convert to destructed refactoring not enabled in JavaScript? | <!-- π¨ STOP π¨ π¦π§π’π£ π¨ πΊπ»πΆπ· π¨
Half of all issues filed here are duplicates, answered in the FAQ, or not appropriate for the bug tracker. Even if you think you've found a *bug*, please read the FAQ first, especially the Common "Bugs" That Aren't Bugs section!
Please help us by doing the following steps before logging an issue:
* Search: https://github.com/Microsoft/TypeScript/search?type=Issues
* Read the FAQ: https://github.com/Microsoft/TypeScript/wiki/FAQ
Please fill in the *entire* template below.
-->
<!-- Please try to reproduce the issue with `typescript@next`. It may have already been fixed. -->
**TypeScript Version:** 3.5.0-dev.20190404
<!-- Search terms you tried before logging this (so others can find this issue more easily) -->
**Search Terms:**
- refactor / refactoring
- convert to destructed parameters
**Code**
For a simple js file
```js
function doStuff(a, b, c) {
return a + b + c;
}
doStuff('a', 'b', 1);
doStuff('x', 'y', 2);
```
**Expected behavior:**
A convert to restructured refactoring is available on `doStuff`
**Actual behavior:**
I'm not seeing any such refactoring show up in js files
**Playground Link:** <!-- A link to a TypeScript Playground "Share" link which demonstrates this behavior -->
**Related Issues:** <!-- Did you find other bugs that looked similar? -->
- #23552
| Suggestion,In Discussion,Domain: Refactorings,Domain: JavaScript | low | Critical |
429,549,111 | vscode | Default formatter selection should write to project specific settings rather than global. | The new Default formatter selection feature, while handy, is unusable for it's stated usecase.
From the [updates page](https://code.visualstudio.com/updates/v1_33) "Many of our users work on different projects and often different projects use different document formatters." Right now, the quick pick will write to the user settings.json, which means every project the particular user works on will share a formatter, and to use it for the usecase of *different* projects using *different* formatters you would have to manually move it to the project local `.vscode/settings.json` directory.
It should also do this for workspace specific settings. | help wanted,feature-request,formatting | low | Minor |
429,586,569 | flutter | Space between text and underline | Internal: b/165577829
I'm using Text.rich with underlined option to make underlined text. It works, but it's possible to add space between the word and underline line? Or make the line more bolder ? | c: new feature,a: typography,customer: crowd,P2,team-engine,triaged-engine | low | Critical |
429,595,192 | go | cmd/go: go test ./... gives duplicate reports when no source files availabe | <!-- Please answer these questions before submitting your issue. Thanks! -->
### What version of Go are you using (`go version`)?
<pre>
% go version
go version devel +964fe4b80f Thu Apr 4 00:26:24 2019 +0000 darwin/amd64
</pre>
### Does this issue reproduce with the latest release?
yes
### What did you do?
<!--
If possible, provide a recipe for reproducing the error.
A complete runnable program is good.
A link on play.golang.org is best.
-->
```
% mkdir ~/devel/test && cd ~/devel/test
% go mod init github.com/davecheney/test
% go test ./...
```
### What did you expect to see?
```
no packages to test
```
*or*
```
"./..." matched no packages
```
*or*
no output
### What did you see instead?
```
% go test ./...
go: warning: "./..." matched no packages
no packages to test
```
Note the duplicate warning and error message. | NeedsInvestigation,GoCommand | low | Critical |
429,596,144 | pytorch | [c10d] CUDA tests for C++ reducer | This requires a split between multi process tests and single process tests for the c10d tests. We cannot use CUDA in the main process, or it will interfere with the multi process tests (that use multiprocessing and the fork spawn method).
There might be an out where we mix and match by using a different start method, but this is not supported equally well across Python versions and/or platforms (from what I remember there are Python 3 specific changes to multiprocessing start methods). | oncall: distributed,triaged | low | Minor |
429,619,264 | pytorch | Completion of error handling | Would you like to [add more error handling](https://github.com/pytorch/pytorch/blob/792cb774f152bab5b968f4ec51da0fc21ff9e895/aten/src/THC/THCGeneral.cpp#L57 "Update candidate: THCudaInit()") for return values from [functions like βcallocβ](https://pubs.opengroup.org/onlinepubs/9699919799/functions/calloc.html "Memory allocation")? | module: internals,low priority,module: error checking,triaged | low | Critical |
429,650,296 | godot | Loader `ResourceFormatLoaderDynamicFont` actually loads type `DynamicFontData` | **Godot version:**
3.1.stable.official
**OS/device including version:**
All
**Issue description:**
Loader `ResourceFormatLoaderDynamicFont` actually loads type `DynamicFontData`.
This seems like either a naming, documentation or API issue (thus related to #24255):
* One "fix" option is to rename `ResourceFormatLoaderDynamicFont` to `ResourceFormatLoaderDynamicFontData`.
* But from my perspective, for a "more useful" API it would be better to change `ResourceFormatLoaderDynamicFont` to actually create a `DynamicFont` instance with a default size using the DynamicFontData loaded from the supplied font path.
However I realise this may conflict with the existing API/implementation.
Currently, the most compact correct way to use a `.ttf` file directly via code seems to be:
```
var dynamic_font = DynamicFont.new()
dynamic_font.font_data = load("res://fonts/barlow/BarlowCondensed-Bold.ttf")
dynamic_font.size = 64
$"CenterContainer/Label".set("custom_fonts/font", dynamic_font)
```
**Steps to reproduce:**
Example code snippet (assuming the label and `.ttf` font file exist):
```
$"CenterContainer/Label".set("custom_fonts/font", load("res://fonts/barlow/BarlowCondensed-Bold.ttf"))
```
Which results in this error:
```
E 0:00:01:0013 Condition ' p_font.is_null() ' is true.
<C Source> scene/gui/control.cpp:1837 @ add_font_override()
<Stack Trace> Root.gd:83 @ _ready()
```
The related code for the resource loading is here, where it can be seen that the resource loader name and type name are not equivalent:
https://github.com/godotengine/godot/blob/a18989602b00f2940befa0b780af14efc7603dd8/scene/resources/dynamic_font.cpp#L1114-L1146
| enhancement,discussion,topic:core | low | Critical |
429,668,256 | pytorch | Caffe2 on google colab: | Dear caffe2 / pytorch afficionados,
I would much appreciate any help on the problem listed below!
I have to add that the script I am providing that can reproduce the error was the result of me trying to get densepose (using caffe2) to work for quite some time in a trial and error style -- because I am very much a greenhorn with python / shell scripting etc. It finally worked, but all of a sudden it stopped to work (some time between the 23rd of March and the 2nd of April).
So if you think that the way I set up densepose in this script intended to be run on google colab is overly complicated and have ideas how to simplify it, that would be very much appreciated as well :) !
I have also found this notebook
https://github.com/Dene33/Detectron/blob/master/notebooks/detectron(google_colab).ipynb
which is supposed to set up caffe2 on google colab, however, it fails when I try it.
So, here's my bug:
## π Bug
When I install caffe2 on a colab instance, I get the following error in response to these commands:
!python2 -c 'from caffe2.python import workspace; print(workspace.NumCudaDevices())'
!python2 -c 'from caffe2.python import core' 2>/dev/null && echo "Success" || echo "Failure"
WARNING:root:This caffe2 python run does not have GPU support. Will run in CPU only mode.
/bin/bash: line 1: 1612 Segmentation fault (core dumped) python2 -c 'from caffe2.python import core' 2> /dev/null
Failure
The very same code used to work, the last time that it ran successfully was on the 23rd of March 2019.
The runtime has GPU enabled.
## To Reproduce
You can run my code in a colab instance:
https://github.com/cdaube/dance/blob/master/setUpDensePose.ipynb
## Expected behavior
I expected this line:
!python2 -c 'from caffe2.python import workspace; print(workspace.NumCudaDevices())'
to not report the missing GPU support,
and I expected this line
!python2 -c 'from caffe2.python import core' 2>/dev/null && echo "Success" || echo "Failure"
to echo "Success"
Many many thanks,
Christoph | caffe2 | low | Critical |
429,673,692 | flutter | Allow multiline text in AppBar | It seems that AppBar does not allow a flexible AppBarHeight depending on its title's content.
In the material guidelines, an AppBar with multiline text is shown as a possible AppBar variant, yet it doesn't seem like Flutter supports this use case. A [question on StackOverflow](https://stackoverflow.com/questions/50764558/expand-the-app-bar-in-flutter-to-allow-multi-line-title) has been asked about this, workarounds are suggested, but each one has drawbacks and uses widgets not really meant for this use case. | c: new feature,framework,f: material design,c: proposal,P3,team-design,triaged-design | medium | Major |
429,687,863 | tensorflow | Unexpected UnicodeDecodeError: invalid continuation byte when reading lines from a file | **System information**
- Have I written custom code (as opposed to using a stock example script provided in TensorFlow): yes
- OS Platform and Distribution (e.g., Linux Ubuntu 16.04): Ubuntu 16.04.5 LTS
- Mobile device (e.g. iPhone 8, Pixel 2, Samsung Galaxy) if the issue happens on mobile device: n/a
- TensorFlow installed from (source or binary): binary
- TensorFlow version (use command below): 1.13.1
- Python version: 3.5.2
- Bazel version (if compiling from source): n/a
- GCC/Compiler version (if compiling from source): n/a
- CUDA/cuDNN version: n/a
- GPU model and memory: n/a
**Describe the current behavior**
Unexpected and undocumented runtime exception/error when handling malformed data.
**Describe the expected behavior**
Expected a "TypeError" or an empty list as a result.
**Code to reproduce the issue**
```
import csv
import sys
import tensorflow as tf
input_file_name = sys.argv[1]
with tf.gfile.Open(input_file_name, "r") as f:
reader = csv.reader(f, delimiter="\t", quotechar=None)
for line in reader:
print(line)
```
Run with the path to the attached file as a command line argument.
**Other info / logs**
Traceback (most recent call last):
File "tensorflow_bug.py", line 9, in <module>
for line in reader:
File "/usr/local/lib/python3.5/dist-packages/tensorflow/python/lib/io/file_io.py", line 220, in \_\_next\_\_
return self.next()
File "/usr/local/lib/python3.5/dist-packages/tensorflow/python/lib/io/file_io.py", line 214, in next
retval = self.readline()
File "/usr/local/lib/python3.5/dist-packages/tensorflow/python/lib/io/file_io.py", line 184, in readline
return self._prepare_value(self._read_buf.ReadLineAsString())
File "/usr/local/lib/python3.5/dist-packages/tensorflow/python/lib/io/file_io.py", line 100, in _prepare_value
return compat.as_str_any(val)
File "/usr/local/lib/python3.5/dist-packages/tensorflow/python/util/compat.py", line 107, in as_str_any
return as_str(value)
File "/usr/local/lib/python3.5/dist-packages/tensorflow/python/util/compat.py", line 80, in as_text
return bytes_or_text.decode(encoding)
UnicodeDecodeError: 'utf-8' codec can't decode byte 0xed in position 0: invalid continuation byte
[corrupted_file1.zip](https://github.com/tensorflow/tensorflow/files/3047460/corrupted_file1.zip)
| stat:awaiting tensorflower,type:bug,comp:ops,TF 2.11 | low | Critical |
429,785,488 | vscode | Mac shortcut not working (in vscode) [cmd+h or cmd+m] | <!-- Please search existing issues to avoid creating duplicates. -->
<!-- Also please test using the latest insiders build to make sure your issue has not already been fixed: https://code.visualstudio.com/insiders/ -->
<!-- Use Help > Report Issue to prefill these. -->
- VSCode Version: 1.60.1 ( Recent )
- OS Version: Mac 11.15.2
Steps to Reproduce:
1. On Release Note Screen
2. (hide or minimize) `cmd+h` or `cmd+m` does not work (have not tried other shortcut).
<!-- Launch with `code --disable-extensions` to check. -->
Does this issue occur when all extensions are disabled?: Yes
Issue still exist (Jan 16, 2025)
```
Version: 1.96.2 (Universal)
Commit: fabdb6a30b49f79a7aba0f2ad9df9b399473380f
Date: 2024-12-19T10:22:47.216Z
Electron: 32.2.6
ElectronBuildId: 10629634
Chromium: 128.0.6613.186
Node.js: 20.18.1
V8: 12.8.374.38-electron.0
OS: Darwin arm64 24.2.0
```
| bug,help wanted,webview | high | Critical |
429,814,983 | go | testing: exclude statically unreachable code in test coverage | <!-- Please answer these questions before submitting your issue. Thanks! -->
### What version of Go are you using (`go version`)?
<pre>
$ go version
go version go1.12.1 darwin/amd64
</pre>
### Does this issue reproduce with the latest release?
yes
### What operating system and processor architecture are you using (`go env`)?
<details><summary><code>go env</code> Output</summary><br><pre>
$ go env
GOARCH="amd64"
GOBIN=""
GOCACHE="/Users/santhosh/Library/Caches/go-build"
GOEXE=""
GOFLAGS=""
GOHOSTARCH="amd64"
GOHOSTOS="darwin"
GOOS="darwin"
GOPATH="/Users/santhosh/go"
GOPROXY=""
GORACE=""
GOROOT="/usr/local/go"
GOTMPDIR=""
GOTOOLDIR="/usr/local/go/pkg/tool/darwin_amd64"
GCCGO="gccgo"
CC="clang"
CXX="clang++"
CGO_ENABLED="1"
GOMOD=""
CGO_CFLAGS="-g -O2"
CGO_CPPFLAGS=""
CGO_CXXFLAGS="-g -O2"
CGO_FFLAGS="-g -O2"
CGO_LDFLAGS="-g -O2"
PKG_CONFIG="pkg-config"
GOGCCFLAGS="-fPIC -m64 -pthread -fno-caret-diagnostics -Qunused-arguments -fmessage-length=0 -fdebug-prefix-map=/var/folders/v5/44ncgqws3dg3pmjnktpt_l2w0000gq/T/go-build063045597=/tmp/go-build -gno-record-gcc-switches -fno-common"
</pre></details>
i have code like this in my project:
~~~go
const trace = false
func doSomething() {
if trace {
fmt.Println("some information")
}
}
~~~
because `trace` is `false`, the coverage reports the tracing code as `not covered`
can such code be shown as "not tracked"
I enable `trace` if we are debugging some issue in our code using build tags
I can enable trace during coverage generation, but tracing output is large and it takes significant time.
I have seen `types` package from stdlib also uses `if trace`
so may be this can be taken as enhancement | NeedsDecision,FeatureRequest | high | Critical |
429,818,770 | go | x/text/unicode/norm: tests run over 10 minutes and time out on js/wasm | What's so hard about x/text/unicode/norm that makes it kill js/wasm?
https://build.golang.org/log/275a5abfdca723fd4574927bd1e076ee93e04ee8
```
...
ok golang.org/x/text/search 7.146s
? golang.org/x/text/secure [no test files]
ok golang.org/x/text/secure/bidirule 11.127s
ok golang.org/x/text/secure/precis 13.066s
ok golang.org/x/text/transform 13.374s
? golang.org/x/text/unicode [no test files]
ok golang.org/x/text/unicode/bidi 10.438s
ok golang.org/x/text/unicode/cldr 11.382s
*** Test killed with quit: ran too long (10m0s).
FAIL golang.org/x/text/unicode/norm 600.299s
ok golang.org/x/text/unicode/rangetable 32.598s
ok golang.org/x/text/unicode/runenames 11.347s
ok golang.org/x/text/width 9.961s
```
/cc @neelance @cherrymui @mpvl | Testing,Builders,NeedsInvestigation,arch-wasm | medium | Major |
429,829,878 | rust | std::PathBuff::join("/some/path") overrides the original path in the resulting PathBuf | When join's argument start's with "/" then join overrides any path present in &self making this code valid:
```
let foo = PathBuf::new("/hello");
assert_eq!(foo.join("/world"), PathBuf::new("/world));
```
This is not documented and generates a lot of confusion. | C-enhancement,T-libs-api,A-io | low | Major |
429,842,239 | flutter | Android Device will restart if you try to create a huge platform view | We should handle Surface out of resource exceptions more gracefully. | platform-android,framework,a: platform-views,dependency: android,perf: memory,P3,c: fatal crash,team-android,triaged-android | low | Minor |
429,843,166 | go | x/tools/imports: permission denied causes non-deterministic updating of imports | ### What version of Go are you using (`go version`)?
<pre>
$ go version
go version go1.11.4 linux/amd64
</pre>
### Does this issue reproduce with the latest release?
Yes
### What operating system and processor architecture are you using (`go env`)?
<details><summary><code>go env</code> Output</summary><br><pre>
$ go env
GOARCH="amd64"
GOBIN=""
GOCACHE="/home/ubuntu/.cache/go-build"
GOEXE=""
GOFLAGS=""
GOHOSTARCH="amd64"
GOHOSTOS="linux"
GOOS="linux"
GOPATH="/home/ubuntu/go"
GOPROXY=""
GORACE=""
GOROOT="/usr/local/go"
GOTMPDIR=""
GOTOOLDIR="/usr/local/go/pkg/tool/linux_amd64"
GCCGO="gccgo"
CC="gcc"
CXX="g++"
CGO_ENABLED="1"
GOMOD=""
CGO_CFLAGS="-g -O2"
CGO_CPPFLAGS=""
CGO_CXXFLAGS="-g -O2"
CGO_FFLAGS="-g -O2"
CGO_LDFLAGS="-g -O2"
PKG_CONFIG="pkg-config"
GOGCCFLAGS="-fPIC -m64 -pthread -fmessage-length=0 -fdebug-prefix-map=/tmp/go-build277105160=/tmp/go-build -gno-record-gcc-switches"
</pre></details>
### What did you do?
<!--
If possible, provide a recipe for reproducing the error.
A complete runnable program is good.
A link on play.golang.org is best.
-->
1. Have a project with one directory with code with a missing import, this import is vendored.
2. Have another directory that is owned by root (non-readable by the current user)
3. Run imports.Process
### What did you expect to see?
Consistently see the import added to the file
### What did you see instead?
The import is sometime added and sometimes not added.
### What I think is happening
The problem appears to be that `addExternalCandidates` sometimes sees the package in the vendor dir and sometimes doesn't.
https://github.com/golang/tools/blob/d996c1aa53b10c3c47e1764d087a569bb6c32ed1/imports/fix.go#L682
I think it's because it calls `gopathResolver.scan` to find a list of all packages
https://github.com/golang/tools/blob/d996c1aa53b10c3c47e1764d087a569bb6c32ed1/imports/fix.go#L910
and scan will quit upon the first error it encounters because it uses `fastwalk.Walk` https://github.com/golang/tools/blob/923d25813098b2ffdf1b4bb7ee4ec425fef796a9/internal/fastwalk/fastwalk.go#L195
Because it quits on the first error, if it reads the vendor dir with the wanted import first, then the import will be there, and if it hits the dir it can't read first, then it will exit without the import.
I think we should always walk the entire tree and walk around unreadable directories rather than aborting the entire walk at that point.
I can see 2 options:
1. Change behavior to gathering errors and keep walking the entire tree
2. Add configuration options to the walker that allows for multiple walk behaviors (abort on first error, or gather errors)
I'm happy to help with making this change as well.
### Related Issue(s)
This issue appears similar to https://github.com/golang/go/issues/16890 but it seems like that question is more targetted towards what log messages are printed, here I'm more concerned about the actual behavior (which dirs are scanned). | NeedsInvestigation,Tools | low | Critical |
429,847,687 | vscode | [css] highlighting not working for some transition properties | As per title - CSS syntax highlighting is not working for some [animatable properties](https://developer.mozilla.org/en-US/docs/Web/CSS/CSS_animated_properties) when using CSS `transition:`, such as `background`, `background-color`, `border-bottom` and probably many more.
<!-- Please search existing issues to avoid creating duplicates. -->
<!-- Also please test using the latest insiders build to make sure your issue has not already been fixed: https://code.visualstudio.com/insiders/ -->
<!-- Use Help > Report Issue to prefill these. -->
- VSCode Version: 1.33.0 & 1.34.0
- OS Version: macOS 10.14.4
Steps to Reproduce:
1. Use HTML file with linked external CSS file.
2. Use the CSS `transition:` property to target some [animatable properties](https://developer.mozilla.org/en-US/docs/Web/CSS/CSS_animated_properties)
Example (lack of) syntax highlighting...
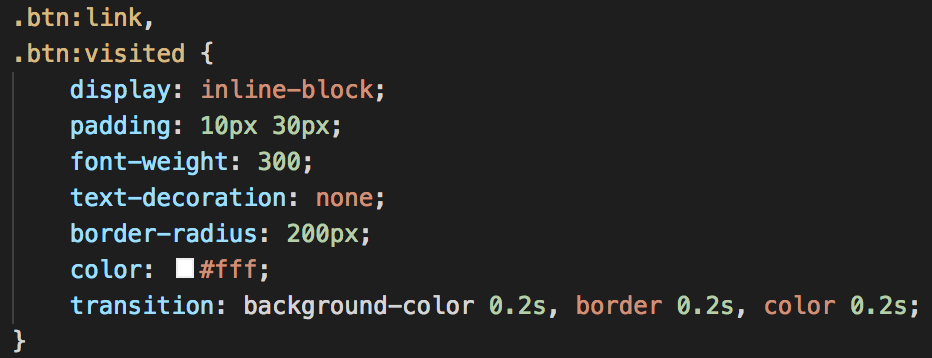
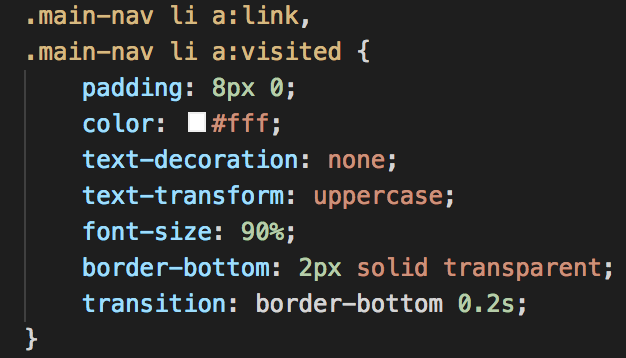
<!-- Launch with `code --disable-extensions` to check. -->
Does this issue occur when all extensions are disabled?: Yes
This issue also seems to exist in other code editors, such as Atom and Brackets, but I've been advised to open an issue here nonetheless.
*edit* *The issue DOES NOT occur in sublime text. | bug,css-less-scss,grammar | low | Major |
429,857,100 | TypeScript | Feature Request: expose getPromisedTypeOfPromise | ## Search Terms
* getPromisedTypeOfPromise
* detect Promise
## Suggestion
expose [`getPromisedTypeOfPromise`](https://github.com/Microsoft/TypeScript/blob/ca98a50574f67d179ee28857b564a086a8f29197/src/compiler/types.ts#L3089) in the `TypeChecker` API.
## Use Cases
Writing lint rules with typechecking involving promises. One example is one checking the result of a yield in a generator. Since yields inside generators are not typed, being able to use linting to check types are properly annotated can be powerful.
## Examples
```
// source code
const myAsyncFunc = async (num: number) => num;
function* gen() {
const y = yield call(myFunc);
}
```
`call` is a redux saga effect that has an API contract of calling a passed function and awaiting if it is a promise. Given this contract, its possible to write a lint rule instructing a consumer to annotate `y` with `: number`.
```
// lint rule
...
let returnType = appliedFunctionType.getCallSignatures()[0].getReturnType();
if(// is promise) {
returnType = typeChecker.getPromisedTypeOfPromise(returnType);
}
```
## Checklist
My suggestion meets these guidelines:
* [x] This wouldn't be a breaking change in existing TypeScript/JavaScript code
* [x] This wouldn't change the runtime behavior of existing JavaScript code
* [x] This could be implemented without emitting different JS based on the types of the expressions
* [x] This isn't a runtime feature (e.g. library functionality, non-ECMAScript syntax with JavaScript output, etc.)
* [x] This feature would agree with the rest of [TypeScript's Design Goals](https://github.com/Microsoft/TypeScript/wiki/TypeScript-Design-Goals).
| API,Needs Investigation | low | Minor |
429,882,470 | rust | Lifetime mismatch on BTreeMap's lookup key | The following code:
struct MyMap(BTreeMap<Cow<'static,str>, Vec<u8>>);
impl MyMap {
fn get(&self, c: &Cow<str>) -> Option<&Vec<u8>> {
self.0.get(c)
}
}
Fails to compile with the following error message:
error[E0623]: lifetime mismatch
this parameter and the return type are declared with different lifetimes...
...but data from `c` is returned here
I'm not sure if this is an error with the compiler, with `BTreeMap` or even `Cow`, but data from `c` is definitely **not** returned, as c is merely used as a lookup key during the call to `BTreeMap::get`.
Rust version: 1.33 stable and 1.35 nightly both fail. | C-enhancement,A-diagnostics,T-compiler | low | Critical |
429,904,561 | go | cmd/compile: ssa debug does not regenerate/update ssa.html | <!-- Please answer these questions before submitting your issue. Thanks! -->
### What version of Go are you using (`go version`)?
<pre>
$ go version
go version devel +db0c524211 Fri Apr 5 15:42:17 2019 +0000 linux/amd64
</pre>
### Does this issue reproduce with the latest release?
yes
### What operating system and processor architecture are you using (`go env`)?
<details><summary><code>go env</code> Output</summary><br><pre>
$ go env
GOARCH="amd64"
GOBIN=""
GOCACHE="/home/cuonglm/.cache/go-build"
GOEXE=""
GOFLAGS=""
GOHOSTARCH="amd64"
GOHOSTOS="linux"
GOOS="linux"
GOPATH="/home/cuonglm/go"
GOPROXY=""
GORACE=""
GOROOT="/home/cuonglm/sources/go"
GOTMPDIR=""
GOTOOLDIR="/home/cuonglm/sources/go/pkg/tool/linux_amd64"
GCCGO="gccgo"
CC="gcc"
CXX="g++"
CGO_ENABLED="1"
GOMOD="/dev/null"
CGO_CFLAGS="-g -O2"
CGO_CPPFLAGS=""
CGO_CXXFLAGS="-g -O2"
CGO_FFLAGS="-g -O2"
CGO_LDFLAGS="-g -O2"
PKG_CONFIG="pkg-config"
GOGCCFLAGS="-fPIC -m64 -pthread -fmessage-length=0 -fdebug-prefix-map=/tmp/go-build070945894=/tmp/go-build -gno-record-gcc-switches"
</pre></details>
### What did you do?
file p.go:
```
package p
func p(slc []byte, i uint) {
if len(slc) >= 3 {
_ = slc[i%3]
}
}
```
```
$ GOSSAFUNC=p GO111MODULE=off go-tip build
# _/home/cuonglm/bin/go/29872
dumped SSA to ./ssa.html
$ cp ssa.html ssa.html.bak
$ diff ssa.html ssa.html | wc -l
0
```
Edit `p.go` to become:
```
package p
func p(slc []byte, i uint) {
i = 1
if len(slc) >= 3 {
_ = slc[i%3]
}
}
```
Then:
```
$ GOSSAFUNC=p GO111MODULE=off go-tip build
# _/home/cuonglm/bin/go/29872
dumped SSA to ./ssa.html
$ diff ssa.html ssa.html.bak | wc -l
6
```
Seems good. Change `p.go` to original content, mean remove line `i = 1`. Then:
```
$ GOSSAFUNC=p GO111MODULE=off go-tip build
# _/home/cuonglm/bin/go/29872
dumped SSA to ./ssa.html
# Here the content of ssa.html and ssa.html.bak must be the same
# but they don't.
$ diff ssa.html ssa.html.bak | wc -l
6
$ rm ssa.html
$ GOSSAFUNC=p GO111MODULE=off go-tip build
# _/home/cuonglm/bin/go/29872
dumped SSA to ./ssa.html
$ ls
p.go ssa.html.bak
```
### What did you expect to see?
`ssa.html` content always update, and file is re-created after deleting.
### What did you see instead?
Content never updates from 3rd run, file is not re-created after deleting. | NeedsInvestigation | low | Critical |
429,920,262 | node | readable.push always returning false after reaching highWaterMark for the first time | <!--
Thank you for reporting a possible bug in Node.js.
Please fill in as much of the template below as you can.
Version: output of `node -v`
Platform: output of `uname -a` (UNIX), or version and 32 or 64-bit (Windows)
Subsystem: if known, please specify the affected core module name
If possible, please provide code that demonstrates the problem, keeping it as
simple and free of external dependencies as you can.
-->
According to the documentation and multiple posts regarding `Readable` streams, `.push` will return false once `highWaterMark` is reached, at that moment `_read` should be stopped.
The issue I'm facing, and I'm doubting whether I misunderstood something or there's an actual bug, probably the former, is that after 16384 bytes are pushed, and `.push` returns false for the first time, all remaining calls to `.push` are returning `false` (9983616 in total).
```javascript
const { Readable } = require('stream');
const writeStream = fs.createWriteStream('./bigFile.txt');
let readCalls = 0;
class CustomReader extends Readable {
constructor(opt) {
super(opt);
this._max = 1e7;
this._index = 0;
}
_read(size) {
readCalls++;
while (this._index < this._max) {
this._index++
if (this._index >= this._max)
return this.push(null);
if (!this.push('a'))
return;
}
}
}
console.time('read');
new CustomReader().pipe(writeStream)
.on('finish', () => {
console.log(readCalls);
console.timeEnd('read');
});
```
In that example, I expect: `readCalls` to be `~610` (`1e7 / 16384`), but instead is `9983616`.
Also I expect it to run in almost the same time than the following script using `.write`
```javascript
(async() => {
console.time('write');
const file = fs.createWriteStream('./test.dat');
for (let i = 0; i < 1e7; i++) {
if (!file.write('a')) {
await new Promise(resolve => file.once('drain', resolve));
}
}
console.timeEnd('write');
})();
```
But it's taking twice the time, which is logical since `_read` is being called a lot more times than it should.
If I completely ignore the return value from `.push` and only depend on `size` argument, the Stream work as expected.
```javascript
class CustomReader extends Readable {
constructor(opt) {
super(opt);
this._max = 1e7;
this._index = 0;
}
_read(size) {
readCounter++;
const stop = Math.min(this._index + size, this._max);
while (this._index < stop) {
this._index++
if (this._index >= this._max)
return this.push(null);
if (!this.push('a')) {
// ignore
// return;
}
}
}
}
// readCounter = 611
```
So the question is, after `.push` returns `false`, besides from stopping the `_read` function, should I wait for a specific event or do an extra check inside `_read`? If not, is this the expected behaviour?
* **Version**: 10.15.3 / 8.15.0
* **Platform**: Ubuntu 18:04
* **Subsystem**:
<!-- Please provide more details below this comment. -->
| stream | medium | Critical |
429,973,852 | pytorch | Tracing and Scripting nn.Conv2d shows different op in the graph | > I have a simple model with nn.Conv2d. When i trace, graphs shows below aten op
>%input : Double(1, 20, 24, 24) = aten::_convolution(%input.1, %1, %2, %5, %8, %11, %12, %15, >%16, %17, %18, %19), scope: testModule/Conv2d[conv1]
>When using script front end, it shows :
> %274 : Tensor = aten::conv2d(%ret, %1, %2, %272, %271, %273, %21)
This happens because in variableType we only record the trace for `aten::_convolution` but not any other conv ops like `aten::conv2d` etc.
I think it's better for us to make scripting and tracing frontend more consistent in ops that emit so that we could do optimizations together to both. | oncall: jit | low | Minor |
429,979,874 | go | net: Listen always uses nextPort() instead of the desired port on JS | <!-- Please answer these questions before submitting your issue. Thanks! -->
### What version of Go are you using (`go version`)?
<pre>
$ go version
go version go1.12.1 darwin/amd64
</pre>
Since this bug has to do with compiling to WebAssembly and running in Node.js, here is my Node.js version too:
<pre>
$ node --version
v11.7.0
</pre>
### Does this issue reproduce with the latest release?
Yes.
### What operating system and processor architecture are you using (`go env`)?
<details><summary><code>go env</code> Output</summary><br><pre>
GOARCH="amd64"
GOBIN=""
GOCACHE="/Users/alex/Library/Caches/go-build"
GOEXE=""
GOFLAGS=""
GOHOSTARCH="amd64"
GOHOSTOS="darwin"
GOOS="darwin"
GOPATH="/Users/alex/programming/go"
GOPROXY=""
GORACE=""
GOROOT="/Users/alex/.go"
GOTMPDIR=""
GOTOOLDIR="/Users/alex/.go/pkg/tool/darwin_amd64"
GCCGO="gccgo"
CC="clang"
CXX="clang++"
CGO_ENABLED="1"
GOMOD=""
CGO_CFLAGS="-g -O2"
CGO_CPPFLAGS=""
CGO_CXXFLAGS="-g -O2"
CGO_FFLAGS="-g -O2"
CGO_LDFLAGS="-g -O2"
PKG_CONFIG="pkg-config"
GOGCCFLAGS="-fPIC -m64 -pthread -fno-caret-diagnostics -Qunused-arguments -fmessage-length=0 -fdebug-prefix-map=/var/folders/h1/vhbpm31925nd0whbv4qd8mr00000gn/T/go-build857099932=/tmp/go-build -gno-record-gcc-switches -fno-common"
</pre></details>
However, it's worth noting that the bug only occurs when you set `GOOS=js GOARCH=wasm`.
### What did you do?
<!--
If possible, provide a recipe for reproducing the error.
A complete runnable program is good.
A link on play.golang.org is best.
-->
Consider the following Go program:
```go
package main
import (
"fmt"
"net"
)
func main() {
ln, err := net.Listen("tcp", "127.0.0.1:8080")
if err != nil {
panic(err)
}
defer ln.Close()
fmt.Println(ln.Addr())
}
```
Run the program with the following command (From the [Go WebAssembly Wiki](https://github.com/golang/go/wiki/WebAssembly)):
```
GOOS=js GOARCH=wasm go run -exec="$(go env GOROOT)/misc/wasm/go_js_wasm_exec" main.go
```
### What did you expect to see?
The program should output `127.0.0.1:8080` since that is the address I passed to `net.Listen`.
If you run the program with `go run main.go` (not compiling to WebAssembly) you see the output that I would expect.
### What did you see instead?
```
127.0.0.1:1
```
I believe the root of the problem is [the `socket` function in __net_fake.go__](https://github.com/golang/go/blob/cb6646234cb6565d19d9efea987c8d8fc9be5c31/src/net/net_fake.go#L65). It always uses `nextPort()` instead of using the port in the `laddr` argument. | NeedsFix,arch-wasm,OS-JS | low | Critical |
429,989,448 | flutter | Texts of different sizes are misaligned at the leading edge of Text widgets due to font spacing | <!-- Thank you for using Flutter!
If you are looking for support, please check out our documentation
or consider asking a question on Stack Overflow:
* https://flutter.dev/
* https://docs.flutter.io/
* https://stackoverflow.com/questions/tagged/flutter?sort=frequent
If you have found a bug or if our documentation doesn't have an answer
to what you're looking for, then fill our the template below. Please read
our guide to filing a bug first: https://flutter.dev/bug-reports/
-->
## Description and file to reproduce
<!--
Please tell us exactly how to reproduce the problem you are running into.
Please attach a small application (ideally just one main.dart file) that
reproduces the problem. You could use https://gist.github.com/ for this.
If the problem is with your application's rendering, then please attach
a screenshot and explain what the problem is.
-->
I'm using the default font, and I have also reproduced this bug after installing Roboto font files from Google.
After creating two Text widgets with two different font sizes in a column (with crossAlignment attribute set to left), it appears that they're not aligned correctly on the left as there seems to be a space before the first letter. As I increased the font size of one of the widgets, this space became more and more visible, leading to a misalignment with the second widget's text.
I'm aware this can be a font problem, but as it can be reproduced with the default Flutter font I don't think this should be a normal behaviour.
Here's an example file to reproduce the problem : https://gist.github.com/axel-op/292fe7af555c9f986068fb44b073e834. I placed the Text widgets in colored containers to see where the edges of the widgets are.
## Logs
<!--
Run your application with `flutter run --verbose` and attach all the
log output below between the lines with the backticks. If there is an
exception, please see if the error message includes enough information
to explain how to solve the issue.
-->
Flutter run --verbose:
Logs can be consulted at this page: https://gist.github.com/axel-op/c796c876be1d5bccab0de36b5c187b4a.
<!--
Run `flutter analyze` and attach any output of that command below.
If there are any analysis errors, try resolving them before filing this issue.
-->
Flutter analyze:
See https://gist.github.com/axel-op/27d15c0134ed2f701505ebb4b1c87967.
<!-- Finally, paste the output of running `flutter doctor -v` here. -->
Flutter doctor -v:
See https://gist.github.com/axel-op/8e83dc27d14d09c420c119d510cbc35d.
| framework,a: typography,has reproducible steps,P2,found in release: 3.7,found in release: 3.8,team-framework,triaged-framework | low | Critical |
430,020,298 | flutter | MaterialPageRoute does not provide parallax animation in CupertinoTabView | ## Steps to Reproduce
```
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
home: CupertinoTabScaffold(
tabBuilder: (context, i) {
switch (i) {
case 0:
return CupertinoTabView(
builder: (context) => CupertinoDemoTab1(),
);
break;
case 1:
return CupertinoTabView(
builder: (context) => CupertinoDemoTab1(),
);
break;
}
},
tabBar: CupertinoTabBar(items: [
BottomNavigationBarItem(
title: Text('Page One'),
icon: Icon(Icons.add),
),
BottomNavigationBarItem(
title: Text('Page Two'),
icon: Icon(Icons.add),
)
]),
),
);
}
}
class CupertinoDemoTab1 extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
body: CustomScrollView(
slivers: <Widget>[
CupertinoSliverNavigationBar(
largeTitle: Text("Camera Demo"),
),
SliverList(
delegate: SliverChildListDelegate([Tab1RowItem()]),
),
],
),
);
}
}
class Tab1RowItem extends StatelessWidget {
@override
Widget build(BuildContext context) {
return CupertinoButton(
onPressed: () async {
Navigator.of(context)
.push(new MaterialPageRoute(builder: (context) => Page2()));
},
child: Text("Navigate"),
);
}
}
class Page2 extends StatelessWidget {
@override
Widget build(BuildContext context) {
return CupertinoPageScaffold(
navigationBar: CupertinoNavigationBar(
middle: Text('page 2'),
),
child: Container(
child: Center(child: Text('Page 2')),
),
);
}
}
```
1. Just click "Navigate"
2. Perform a back swipe and watch the bottom most route have no parallax effect
## Logs
```
[β] Flutter (Channel master, v1.4.11-pre.27, on Mac OS X 10.14.2 18C54, locale en-ZA)
β’ Flutter version 1.4.11-pre.27 at /Users/calvinmuller/iStreet/Mobile/flutter
β’ Framework revision d0f89c1190 (9 hours ago), 2019-04-05 18:55:33 -0700
β’ Engine revision 6bc33b5e14
β’ Dart version 2.2.1 (build 2.2.1-dev.3.0 None)
```
| c: new feature,framework,f: material design,f: cupertino,f: routes,P2,team-design,triaged-design | low | Minor |
430,023,893 | flutter | Enable dart:ui APIs for text measurement in secondary isolates | if use TextPainter.layout in isolate will get a compiler error `native function not found`
I need layout many words and it takes a long time (100ms+), it will block UI thread
What should I do? | c: new feature,framework,engine,a: typography,P2,team-engine,triaged-engine | low | Critical |
430,042,396 | pytorch | Performance issue of unique on CPU | Related: https://github.com/pytorch/pytorch/issues/15804 https://github.com/pytorch/pytorch/issues/18405 https://github.com/pytorch/pytorch/pull/16145 https://github.com/pytorch/pytorch/pull/18648
The performance on GPU has been fully resolved.
Newest benchmark on CPU on a tensor of shape `torch.Size([15320, 2])`:
```python
print(torch.__version__)
%timeit a.unique(dim=0, sorted=True, return_inverse=False)
%timeit a.unique(dim=0, sorted=True, return_inverse=True)
%timeit torch._unique_dim2_temporary_will_remove_soon(a, dim=0, sorted=True, return_inverse=False, return_counts=True)
%timeit torch._unique_dim2_temporary_will_remove_soon(a, dim=0, sorted=True, return_inverse=True, return_counts=True)
```
```
1.1.0a0+13f03a4
60.5 ms Β± 115 Β΅s per loop (mean Β± std. dev. of 7 runs, 10 loops each)
60.5 ms Β± 150 Β΅s per loop (mean Β± std. dev. of 7 runs, 10 loops each)
60.7 ms Β± 170 Β΅s per loop (mean Β± std. dev. of 7 runs, 10 loops each)
60.7 ms Β± 217 Β΅s per loop (mean Β± std. dev. of 7 runs, 10 loops each)
```
```python
print(torch.__version__)
%timeit a.unique(sorted=True, return_inverse=False)
%timeit a.unique(sorted=True, return_inverse=True)
%timeit torch._unique2_temporary_will_remove_soon(a, sorted=True, return_inverse=False, return_counts=True)
%timeit torch._unique2_temporary_will_remove_soon(a, sorted=True, return_inverse=True, return_counts=True)
```
```
1.1.0a0+13f03a4
308 Β΅s Β± 690 ns per loop (mean Β± std. dev. of 7 runs, 1000 loops each)
906 Β΅s Β± 769 ns per loop (mean Β± std. dev. of 7 runs, 1000 loops each)
60 ms Β± 96.9 Β΅s per loop (mean Β± std. dev. of 7 runs, 10 loops each)
60.2 ms Β± 254 Β΅s per loop (mean Β± std. dev. of 7 runs, 10 loops each)
```
cc @VitalyFedyunin @ngimel | module: performance,module: cpu,triaged,module: sorting and selection | low | Major |
430,048,433 | flutter | Optimize RenderTransform where any scale axis == 0 | I noticed this while working on #30522
If you introduce a `Transform.scale` that scales its children to exactly 0 on any axis (as can happen when animating the scale), we still do all the layout and painting work for those children.
Painting probably isn't a ton of work, but layout could very well be. We document `performLayout` as _always needing to layout children_, but I think this case should be an exception for a `RenderTransform`.
The proposed logic would look something like:
- Update the `RenderTransform.transform` setter to check if the scale has gone to or from 0 -if so `markNeedsLayout`
- Update the `RenderTransform.scale` method to check if we're scaling to or from 0 - if so, `markNeedsLayout`
- Update the `RenderTransform.performLayout` to check if the scale == 0 on any axis. If so, fizzle and don't have children layout.
- Update the `RenderTransform.paint` to fizzle if the scale == 0 on any axis.
/cc @goderbauer @Hixie | framework,a: animation,c: proposal,P3,team-framework,triaged-framework | low | Minor |
430,052,062 | youtube-dl | [vlive] ONLY_APP message when downloading vlive+ paid video | ## Please follow the guide below
- You will be asked some questions and requested to provide some information, please read them **carefully** and answer honestly
- Put an `x` into all the boxes [ ] relevant to your *issue* (like this: `[x]`)
- Use the *Preview* tab to see what your issue will actually look like
---
### Make sure you are using the *latest* version: run `youtube-dl --version` and ensure your version is *2019.04.01*. If it's not, read [this FAQ entry](https://github.com/ytdl-org/youtube-dl/blob/master/README.md#how-do-i-update-youtube-dl) and update. Issues with outdated version will be rejected.
- [x] I've **verified** and **I assure** that I'm running youtube-dl **2019.04.01**
### Before submitting an *issue* make sure you have:
- [x] At least skimmed through the [README](https://github.com/ytdl-org/youtube-dl/blob/master/README.md), **most notably** the [FAQ](https://github.com/ytdl-org/youtube-dl#faq) and [BUGS](https://github.com/ytdl-org/youtube-dl#bugs) sections
- [x] [Searched](https://github.com/ytdl-org/youtube-dl/search?type=Issues) the bugtracker for similar issues including closed ones
- [x] Checked that provided video/audio/playlist URLs (if any) are alive and playable in a browser
### What is the purpose of your *issue*?
- [x] Bug report (encountered problems with youtube-dl)
- [ ] Site support request (request for adding support for a new site)
- [x] Feature request (request for a new functionality)
- [ ] Question
- [ ] Other
---
### If the purpose of this *issue* is a *bug report*, *site support request* or you are not completely sure provide the full verbose output as follows:
Add the `-v` flag to **your command line** you run youtube-dl with (`youtube-dl -v <your command line>`), copy the **whole** output and insert it here. It should look similar to one below (replace it with **your** log inserted between triple ```):
```
[debug] System config: []
[debug] User config: []
[debug] Custom config: []
[debug] Command-line args: ['-v', '-u', 'PRIVATE', '-p', 'PRIVATE', 'https://www.vlive.tv/video/54099']
[debug] Encodings: locale cp1252, fs mbcs, out cp850, pref cp1252
[debug] youtube-dl version 2019.04.01
[debug] Python version 3.4.4 (CPython) - Windows-10-10.0.17134
[debug] exe versions: none
[debug] Proxy map: {}
[vlive] 54099: Downloading webpage
ERROR: Unknown status ONLY_APP; please report this issue on https://yt-dl.org/bug . Make sure you are using the latest version; type youtube-dl -U to update. Be sure to call youtube-dl with the --verbose flag and include its complete output.
Traceback (most recent call last):
File "C:\Users\dst\AppData\Roaming\Build archive\youtube-dl\ytdl-org\tmp48z7ci11\build\youtube_dl\YoutubeDL.py", line 794, in extract_info
File "C:\Users\dst\AppData\Roaming\Build archive\youtube-dl\ytdl-org\tmp48z7ci11\build\youtube_dl\extractor\common.py", line 529, in extract
File "C:\Users\dst\AppData\Roaming\Build archive\youtube-dl\ytdl-org\tmp48z7ci11\build\youtube_dl\extractor\vlive.py", line 95, in _real_extract
youtube_dl.utils.ExtractorError: Unknown status ONLY_APP; please report this issue on https://yt-dl.org/bug . Make sure you are using the latest version; type youtube-dl -U to update. Be sure to call youtube-dl with the --verbose flag and include its complete output.
...
<end of log>
```
---
---
### Description of your *issue*, suggested solution and other information
I am unable to download this video (https://www.vlive.tv/video/54099) that I have already paid for.
I think the ONLY_APP message is because it only allow users to download the video using the app but even so, it does not download the actual video as users can only play the video using the app. It would be really great if it is possible to have to download this video in my computer because it always take forever to buffer this video in the website.
Thank you.
| account-needed | low | Critical |
430,067,924 | godot | Y-Billboard Sprite3D does not cast shadows | **Godot version:**
3.06.stable.official.8314054. I had also tried it on 3.1 with the same results.
**OS/device including version:**
Windows 10 Home
NVIDIA GeForce GTX 1080 with Driver Version 25.21.14.1967
**Issue description:**
3D sprites cast shadows when Depth Draw Mode is set to "Opaque Pre-Pass", but when Billboard Mode is set to "Y-Billboard" the sprite no longer casts shadows. Using the normal "Enabled" Billboard Mode works mostly as I expected "Y-Billboard" to also work (shadow is upside down but I think changing properties can fix that).
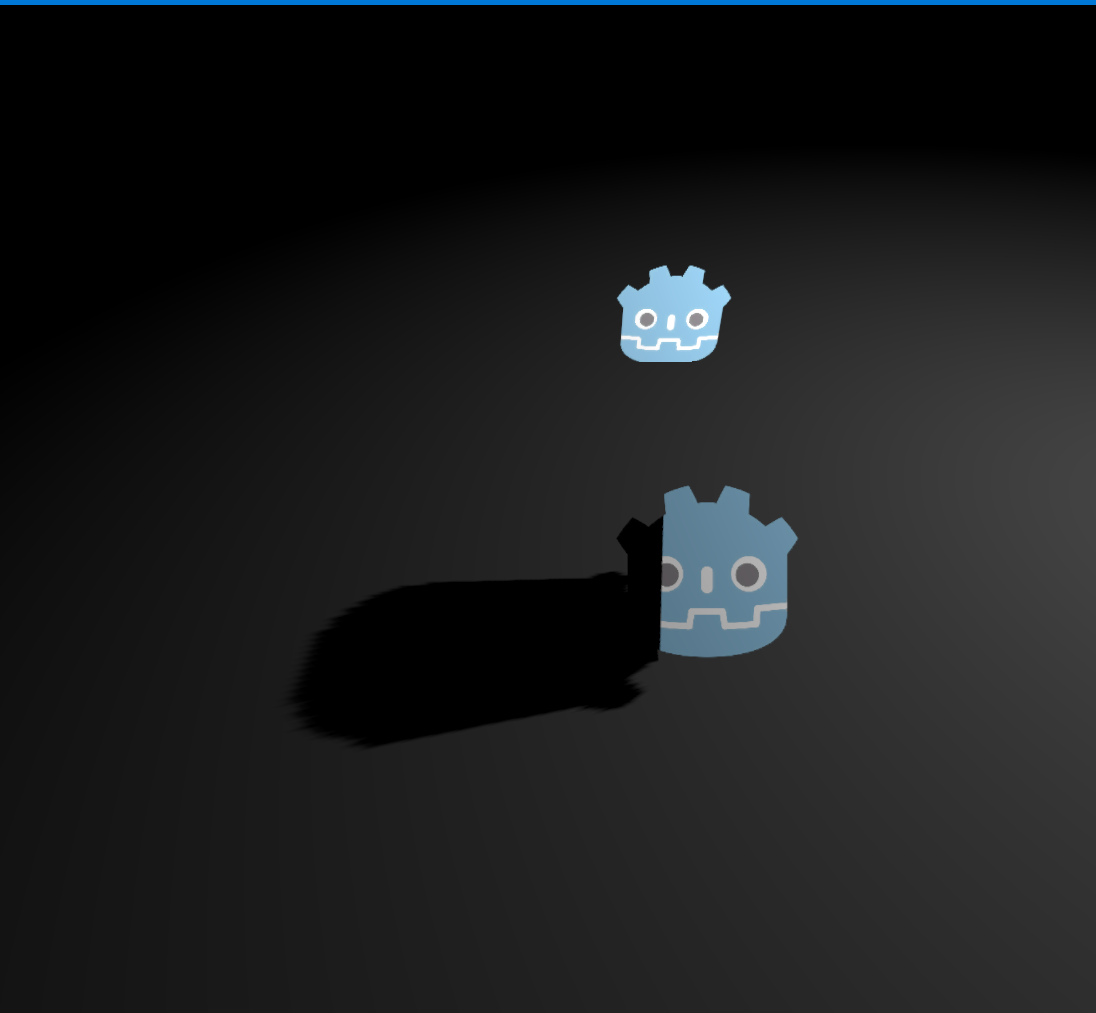
In this example screenshot, the closer sprite is set to "Enabled", and the farther sprite is set to "Y-Billboard". Other properties are the same.
**Steps to reproduce:**
Easiest is to open up the Minimal reproduction project and look at it.
Otherwise get yourself a light, a plane, and a sprite. Set the sprite material Depth Draw Mode to "Opaque Pre-Pass" and set Billboard Mode to "Y-Billboard".
**Minimal reproduction project:**
[Y-Billboard Shadows.zip](https://github.com/godotengine/godot/files/3050841/Y-Billboard.Shadows.zip)
| bug,topic:rendering,confirmed,topic:3d | low | Major |
430,068,163 | go | testing: support explicitly running non-hermetic (or non-deterministic) examples | We can have examples which show up in the godoc but aren't runnable, and runnable examples which are run as part of `go test`.
What about examples which are too slow or unreliable for `go test`, for example those needing access to the internet? I can make them non-runnable, but what if a user wants to give one a try? `go test -run ExampleSlow` doesn't work, and the next best option is copy-pasting into a main func, which is awkward.
The only other option, which is what I tend to do, is to stick examples as main packages elsewhere. But that's also icky; if the example packages aren't hidden, `go install ./...` will include them, and if they're hidden in another repo or in `_examples`, they are hard to find.
Another disadvantage of hiding the examples in a separate dir/repo is that `go test ./...` won't check if they compile fine; one has to remember to check that dir/repo too.
For some context, I'm trying to collapse the https://github.com/chromedp/examples into the main repo, starting with an [example_test.go](https://github.com/chromedp/chromedp/blob/504561eab2c5fb5b2e9b9b88160da2dcf2cfa199/example_test.go) file. The second and third examples are quick, but the first accesses the internet and is slow. I don't want to run it with `go test`, but I want it to show up in godoc and allow people to run it easily to try it out.
I'm not labelling this as a proposal, because I don't have a specific solution in mind; this is just a description of a problem I have when trying to document packages with non-trivial examples.
/cc @acln0 @mpvl @bcmills @ianlancetaylor | NeedsInvestigation | low | Major |
430,069,431 | flutter | Flutter is incompatible with Android Monkey Tests | I've tested the same command with a standard Android app and it works as it should.
## Steps to Reproduce
1. Run the following
```sh
adb shell monkey -p your.package.name --pct-touch 100 -throttle 500 -v 300
```
## Logs
<details><summary>Output</summary>
<p>
:Monkey: seed=1554731839200 count=300
:AllowPackage: dev.materialtime.livelab.mes
:IncludeCategory: android.intent.category.LAUNCHER
:IncludeCategory: android.intent.category.MONKEY
// Event percentages:
// 0: 100.0%
// 1: 0.0%
// 2: 0.0%
// 3: 0.0%
// 4: -0.0%
// 5: -0.0%
// 6: 0.0%
// 7: 0.0%
// 8: 0.0%
// 9: 0.0%
// 10: 0.0%
// 11: 0.0%
:Switch: #Intent;action=android.intent.action.MAIN;category=android.intent.category.LAUNCHER;launchFlags=0x10200000;component=dev.materialtime.livelab.mes/.MainActivity;end
// Allowing start of Intent { act=android.intent.action.MAIN cat=[android.intent.category.LAUNCHER] cmp=dev.materialtime.livelab.mes/.MainActivity } in package dev.materialtime.livelab.mes
:Sending Touch (ACTION_DOWN): 0:(759.0,1709.0)
:Sending Touch (ACTION_UP): 0:(762.0156,1710.8438)
// CRASH: dev.materialtime.livelab.mes (pid 9656)
// Short Msg: java.lang.NullPointerException
// Long Msg: java.lang.NullPointerException: Attempt to invoke virtual method 'float android.view.InputDevice$MotionRange.getMin()' on a null object reference
// Build Label: HUAWEI/CPN/HWCPN-Q:7.0/HUAWEICPN-W09/C128B006:user/release-keys
// Build Changelist: C128B006
// Build Time: 1527839833000
// java.lang.NullPointerException: Attempt to invoke virtual method 'float android.view.InputDevice$MotionRange.getMin()' on a null object reference
// at io.flutter.view.FlutterView.addPointerForIndex(Unknown Source)
// at io.flutter.view.FlutterView.onTouchEvent(Unknown Source)
// at android.view.View.dispatchTouchEvent(View.java:10013)
// at android.view.ViewGroup.dispatchTransformedTouchEvent(ViewGroup.java:2671)
// at android.view.ViewGroup.dispatchTouchEvent(ViewGroup.java:2301)
// at android.view.ViewGroup.dispatchTransformedTouchEvent(ViewGroup.java:2671)
// at android.view.ViewGroup.dispatchTouchEvent(ViewGroup.java:2301)
// at android.view.ViewGroup.dispatchTransformedTouchEvent(ViewGroup.java:2671)
// at android.view.ViewGroup.dispatchTouchEvent(ViewGroup.java:2301)
// at com.android.internal.policy.DecorView.superDispatchTouchEvent(DecorView.java:447)
// at com.android.internal.policy.PhoneWindow.superDispatchTouchEvent(PhoneWindow.java:1871)
// at android.app.Activity.dispatchTouchEvent(Activity.java:3213)
// at com.android.internal.policy.DecorView.dispatchTouchEvent(DecorView.java:409)
// at android.view.View.dispatchPointerEvent(View.java:10233)
// at android.view.ViewRootImpl$ViewPostImeInputStage.processPointerEvent(ViewRootImpl.java:4865)
// at android.view.ViewRootImpl$ViewPostImeInputStage.onProcess(ViewRootImpl.java:4726)
// at android.view.ViewRootImpl$InputStage.deliver(ViewRootImpl.java:4258)
// at android.view.ViewRootImpl$InputStage.onDeliverToNext(ViewRootImpl.java:4311)
// at android.view.ViewRootImpl$InputStage.forward(ViewRootImpl.java:4277)
// at android.view.ViewRootImpl$AsyncInputStage.forward(ViewRootImpl.java:4404)
// at android.view.ViewRootImpl$InputStage.apply(ViewRootImpl.java:4285)
// at android.view.ViewRootImpl$AsyncInputStage.apply(ViewRootImpl.java:4461)
// at android.view.ViewRootImpl$InputStage.deliver(ViewRootImpl.java:4258)
// at android.view.ViewRootImpl$InputStage.onDeliverToNext(ViewRootImpl.java:4311)
// at android.view.ViewRootImpl$InputStage.forward(ViewRootImpl.java:4277)
// at android.view.ViewRootImpl$InputStage.apply(ViewRootImpl.java:4285)
// at android.view.ViewRootImpl$InputStage.deliver(ViewRootImpl.java:4258)
// at android.view.ViewRootImpl.deliverInputEvent(ViewRootImpl.java:6690)
// at android.view.ViewRootImpl.doProcessInputEvents(ViewRootImpl.java:6664)
// at android.view.ViewRootImpl.enqueueInputEvent(ViewRootImpl.java:6625)
// at android.view.ViewRootImpl$WindowInputEventReceiver.onInputEvent(ViewRootImpl.java:6819)
// at android.view.InputEventReceiver.dispatchInputEvent(InputEventReceiver.java:192)
// at android.os.MessageQueue.nativePollOnce(Native Method)
// at android.os.MessageQueue.next(MessageQueue.java:356)
// at android.os.Looper.loop(Looper.java:138)
// at android.app.ActivityThread.main(ActivityThread.java:6623)
// at java.lang.reflect.Method.invoke(Native Method)
// at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:942)
// at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:832)
//
** Monkey aborted due to error.
Events injected: 3
:Sending rotation degree=0, persist=false
:Dropped: keys=0 pointers=0 trackballs=0 flips=0 rotations=0
## Network stats: elapsed time=1045ms (0ms mobile, 0ms wifi, 1045ms not connected)
** System appears to have crashed at event 3 of 300 using seed 1554731839200
</p>
</details> | a: tests,c: new feature,platform-android,engine,P2,team-android,triaged-android | low | Critical |
430,085,940 | go | x/net/html: Tokenizer could track Line/Column of token | <!-- Please answer these questions before submitting your issue. Thanks! -->
### What version of Go are you using (`go version`)?
<pre>
$ go version
go version go1.12.1 linux/amd64
</pre>
### Does this issue reproduce with the latest release?
Yes.
### What operating system and processor architecture are you using (`go env`)?
<details><summary><code>go env</code> Output</summary><br><pre>
$ go env
GOARCH="amd64"
GOHOSTARCH="amd64"
GOHOSTOS="linux"
GOOS="linux"
GCCGO="gccgo"
CC="gcc"
CXX="g++"
CGO_ENABLED="1"
CGO_CFLAGS="-g -O2"
CGO_CPPFLAGS=""
CGO_CXXFLAGS="-g -O2"
CGO_FFLAGS="-g -O2"
CGO_LDFLAGS="-g -O2"
PKG_CONFIG="pkg-config"
GOGCCFLAGS="-fPIC -m64 -pthread -fmessage-length=0 -fdebug-prefix-map=/tmp/go-build083125343=/tmp/go-build -gno-record-gcc-switches"
</pre></details>
### What did you do?
I wanted to get the line and column for the current Token, which forced me to fork the package. The key change is adding something like the following to the end of readByte() in token.go:
```go
// Increment the line and column tracker
if x == '\n' {
z.currentLine++
z.currentColumn = 0
} else {
z.currentColumn++
}
```
### What did you expect to see?
I'd like to see a public method on Tokenizer that returns the starting and ending line/column of the current Token. The method could return a new struct with these four values.
### What did you see instead?
There isn't a way to figure out where the token is in the input aside from byte offset. I could feed that byte offset into user code to determine where the line/column is, but then I'd have to parse the input twice and build up that lookup table first. | NeedsInvestigation,FeatureRequest | low | Critical |
430,089,125 | react | useReducer's dispatch should return a promise which resolves once its action has been delivered | (This is a spinoff from [this thread](https://github.com/facebook/react/issues/15240).)
It's sometimes useful to be able to dispatch an action from within an async function, wait for the action to transform the state, and then use the resulting state to determine possible further async work to do. For this purpose it's possible to define a `useNext` hook which returns a promise of the next value:
```js
function useNext(value) {
const valueRef = useRef(value);
const resolvesRef = useRef([]);
useEffect(() => {
if (valueRef.current !== value) {
for (const resolve of resolvesRef.current) {
resolve(value);
}
resolvesRef.current = [];
valueRef.current = value;
}
}, [value]);
return () => new Promise(resolve => {
resolvesRef.current.push(resolve);
});
}
```
and use it like so:
```js
const nextState = useNext(state);
useEffect(() => {
fetchStuff(state);
}, []);
async function fetchStuff(state) {
dispatch({ type: 'START_LOADING' });
let data = await xhr.post('/api/data');
dispatch({ type: 'RECEIVE_DATA', data });
// get the new state after the action has taken effect
state = await nextState();
if (!state.needsMoreData) return;
data = await xhr.post('/api/more-data');
dispatch({ type: 'RECEIVE_MORE_DATA', data });
}
```
This is all well and good, but `useNext` has a fundamental limitation: it only resolves promises when the state _changes_... so if dispatching an action resulted in the same state (thus causing `useReducer` to [bail out](https://reactjs.org/docs/hooks-reference.html#bailing-out-of-a-state-update)), our async function would hang waiting for an update that wasn't coming.
What we _really_ want here is a way to obtain the state after the last dispatch has taken effect, whether or not it resulted in the state changing. Currently I'm not aware of a foolproof way to implement this in userland (happy to be corrected on this point). But it seems like it could be a very useful feature of `useReducer`'s `dispatch` function itself to return a promise of the state resulting from reducing by the action. Then we could rewrite the preceding example as
```js
useEffect(() => {
fetchStuff(state);
}, []);
async function fetchStuff(state) {
dispatch({ type: 'START_LOADING' });
let data = await xhr.post('/api/data');
state = await dispatch({ type: 'RECEIVE_DATA', data });
if (!state.needsMoreData) return;
data = await xhr.post('/api/more-data');
dispatch({ type: 'RECEIVE_MORE_DATA', data });
}
```
## EDIT
Thinking about this a little more, the promise returned from `dispatch` doesn't need to carry the next state, because there are other situations where you want to obtain the latest state too and we can already solve that with a simple ref. The narrowly-defined problem is: we need to be able to wait until after a `dispatch()` has taken affect. So `dispatch` could just return a `Promise<void>`:
```js
const stateRef = useRef(state);
useEffect(() => {
stateRef.current = state;
}, [state]);
useEffect(() => {
fetchStuff();
}, []);
async function fetchStuff() {
dispatch({ type: 'START_LOADING' });
let data = await xhr.post('/api/data');
// can look at current state here too
if (!stateRef.current.shouldReceiveData) return;
await dispatch({ type: 'RECEIVE_DATA', data });
if (!stateRef.current.needsMoreData) return;
data = await xhr.post('/api/more-data');
dispatch({ type: 'RECEIVE_MORE_DATA', data });
}
``` | Type: Feature Request,Component: Hooks | high | Critical |
430,096,815 | pytorch | ninja: build stopped: subcommand failed. | ## π Bug
[446/3104] Building CXX object third_party/fbgemm/CMakeFiles/fbgemm_avx2.dir/src/FbgemmI8DepthwiseAvx2.cc.o
ninja: build stopped: subcommand failed.
Traceback (most recent call last):
File "setup.py", line 719, in <module>
build_deps()
File "setup.py", line 285, in build_deps
build_dir='build')
File "/home/prjs/tmp/pytorch/tools/build_pytorch_libs.py", line 278, in build_caffe2
check_call(ninja_cmd, cwd=build_dir, env=my_env)
File "/root/miniconda3/envs/py35/lib/python3.5/subprocess.py", line 271, in check_call
raise CalledProcessError(retcode, cmd)
subprocess.CalledProcessError: Command '['ninja', 'install']' returned non-zero exit status 1
| module: build,triaged | low | Critical |
430,097,962 | scrcpy | screen rotation | I have a bug on my LG device where the screen on my pc is rotated 90 degrees but still functions as if the touches were not. it is similar to this issue, https://github.com/Genymobile/scrcpy/issues/186
but the reference there doesn't help me.
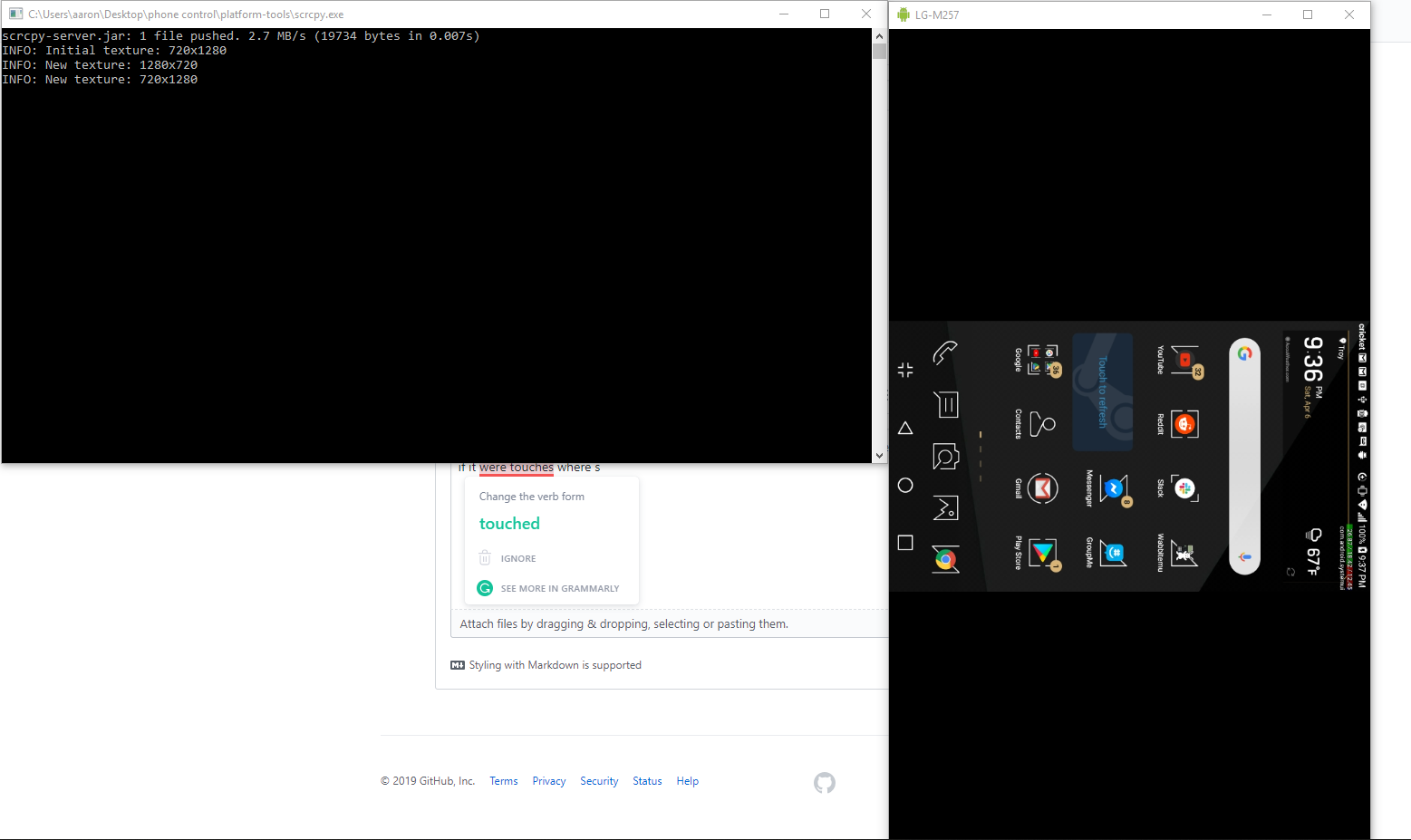
how do i fix this bug and/or rotate the screen on my pc | rotation | low | Critical |
430,127,110 | go | bytes: trivial to beat ContainsAny in easy cases | See this CL: https://go-review.googlesource.com/c/go/+/155965
In short, code like `bytes.ContainsAny(data, " \t")` is easy to read and write, but a simple manual implementation as follows is considerably faster:
```
any := false
for _, b := range data {
if b == ' ' || b == '\t' {
any = true
break
}
}
```
`ContainsAny` uses `IndexAny`, which already has three paths; it does nothing for empty `chars`, it builds an ASCII set if `chars` is all ASCII and over eight bytes, and otherwise it does a naive double-loop search.
I'm not sure what the right solution here would be. I think adding more special cases to `IndexAny` would probably be overkill, and even slow down the other cases because of the added code. Making the compiler treat `ContainsAny` or `IndexAny` in a special way is also undesirable.
I guess if the compiler was super smart in the future, inlined all the code and realised that our chars are just `" \t"`, it would simply inline that last naive search code. But I imagine the current compiler is far away from that possibility.
This is an unfortunate outcome, because the `bytes` and `strings` packages are very intuitive and often the fastest. I'd like to continue relying on them, without having to write my own loops by hand when they start showing up on CPU profiles.
/cc @josharian @randall77 @martisch | Performance,help wanted,NeedsInvestigation | low | Major |
430,139,253 | scrcpy | resize window to 1:1 when rotated | I want to use OBS to capture my phone's screen, and in order to ensure the resolution quality, I press Ctrl+G to make it resize window to 1:1 (pixel-perfect), but the problem is that everytime I rotate my phone, I need to press Ctrl+G again to resize the window. Could you help me to make the window fixed to 1:1 once I press Ctrl+G, thanks. | feature request | low | Minor |
430,141,960 | godot | Improve error reporting when rcedit fails to change icon / set PE data | **Godot version:**
GoDot 3.1 Stable/ rcedit 1.1.1/wine 3.0.2
**OS/device including version:**
Ubuntu 19.04
**Issue description:**
No matter what I try, every time I export for windows .exe file does not have icon. There is no error in log. I tried everything. Using rcedit in terminal gives me error not shown in godot :0009:fixme:ver:GetCurrentPackageId (0x33fe24 (nil)): stub .I'm giving up, I'll just leave here issue in case someone know what to do with this. Nobody knew on discord, nobody on reddit, I don't know why I'm still trying...
| enhancement,platform:windows,topic:editor,topic:porting,good first issue,usability | low | Critical |
430,149,743 | godot | Scale operation doesn't respect that "Use pixel snap" is turned on | **Godot version:**
3.1 stable
**OS/device including version:**
Windows 7 x64
**Steps to reproduce:**
1. Scene editor -> Snapping Options -> "Use Pixel Snap" is turned on,
2. Drag Sprite to Scene editor,
3. Change sprite scale using mouse
**_Result_**: when you change scale this way, sprite position changes too. And this can be half a pixel despite "Use Pixel Snap" option is on.
**_Expected_**: in my opinion it should not behave this way. Scale should get those values which not lead to integer position.
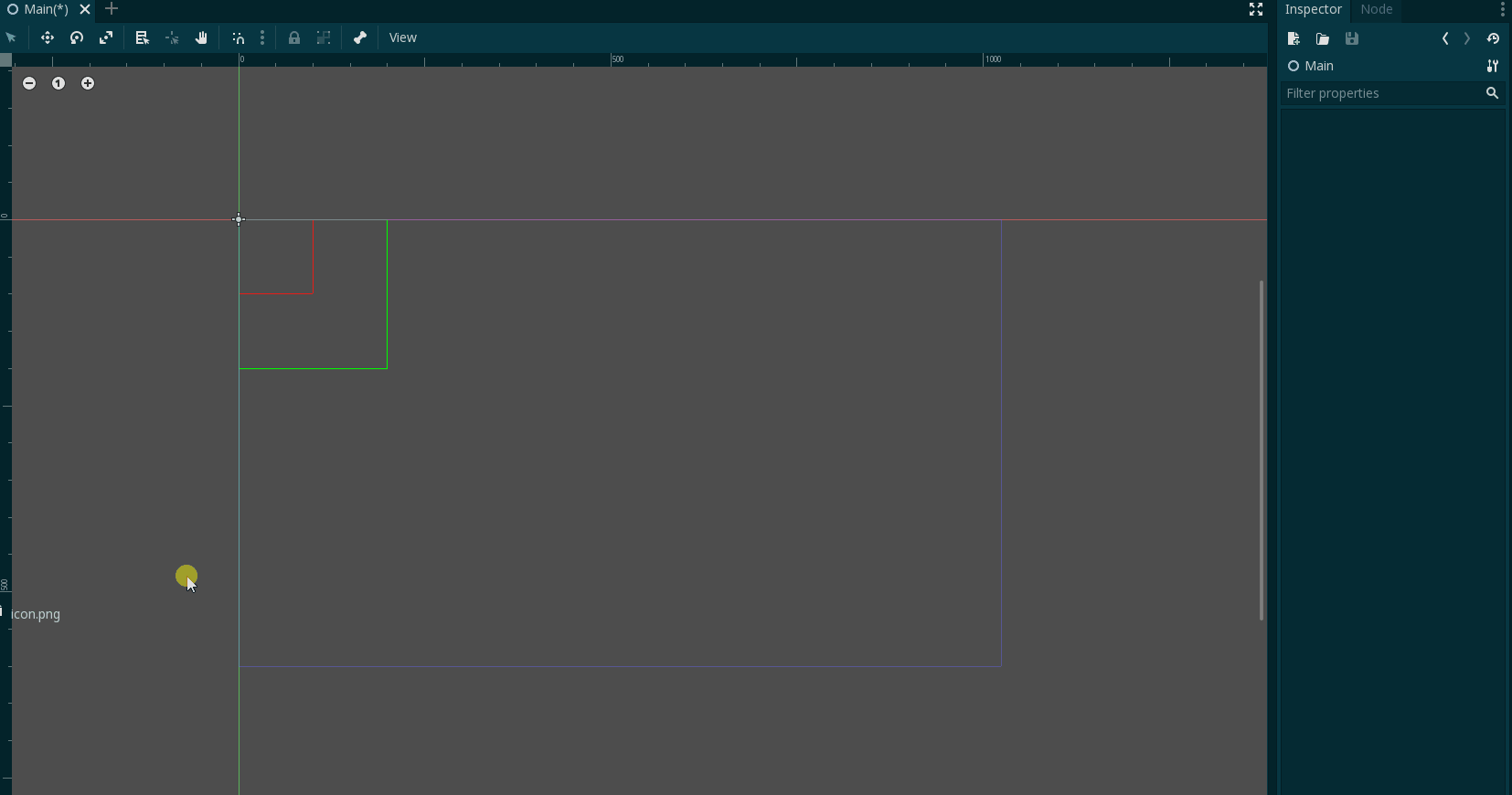
| enhancement,discussion,topic:editor | low | Critical |
430,155,118 | pytorch | torch.from_PIL() Request ? | ## π Feature
A simple method for transforming a PIL images directly into torch tensor.
## Motivation
It's frustrating to use transforms for a simple conversion between a PIL image and torch tensors and in the same time it's very easy to get tensor from numpy through ```torch.from_numpy()```
## Pitch
Giving it a PIL images of any type and it returns a torch tensor
## Alternatives
I have considered two:
1. Transforms
2. convert it first to numpy then to torch
| feature,triaged,module: vision | low | Minor |
430,155,570 | godot | Editor Snap/View settings doesn't saved automatically right after change | **Godot version:**
3.1 stable
**OS/device including version:**
Windows 7 x64
**Steps to reproduce:**
Example:
1. Turn on/off "Snap to grid",
2. Re-open project,
3. Check that "Snap to grid" setting wasn't saved
**_Result_**:
Those settings are saved only when you save the project.
**_Expected_**:
Settings should be saved to file right after change were made, as it is when you change IDE layout or Editor settings.
**Note**:
I guess it's relevant for every settings in "Godot/projects/%project_name%/Main.tscn-editstate-bcb0d2eb5949c52b6a65bfe9de3e985b.cfg" file, like:
snap, view, tileset, texture region, 3D
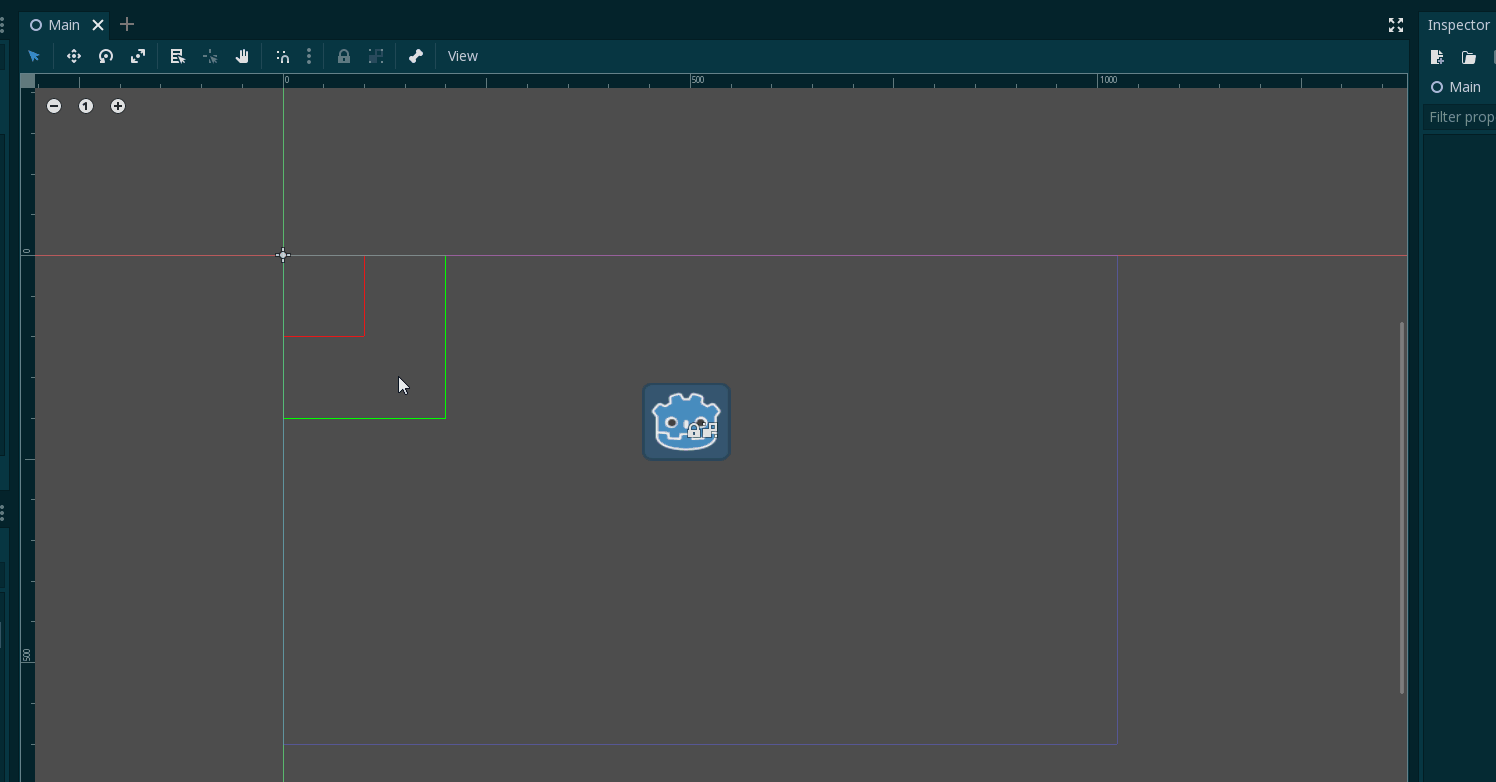
| bug,topic:editor,confirmed | low | Critical |
430,161,205 | rust | Move libtest out of tree | See: https://internals.rust-lang.org/t/a-path-forward-towards-re-usable-libtest-functionality-custom-test-frameworks-and-a-stable-bench-macro
An attempt for moving libtest out of tree was made in https://github.com/rust-lang/rust/pull/57842 , however it broke clippy in weird ways that involve interactions with the rustc build system.
It has been reverted in https://github.com/rust-lang/rust/pull/59766 so that the beta is fixed in time.
We should still work towards making this happen.
A potential plan is to:
- Continue to improve http://github.com/rust-lang/libtest/
- Migrate compiletest-rs to using http://github.com/rust-lang/libtest/, on pure stable
- Make sure rustc compiletest and compiletest-rs match
- Update clippy, miri to use new compiletest-rs, ensure this works within the rustc build system
- Update rustc compiletest to be compiletest-rs (optional, but nice to have)
- Update rustc libtest to reexport libtest
thoughts? @gnzlbg @xales | C-enhancement,T-libs-api,A-libtest | low | Major |
430,162,029 | node | Increase buffer pool size and streams high water marks | Our Buffer pool size is currently set to 8kb. This seems pretty low for most hardware used these days.
Similar with our default high water marks in streams (16kb for all streams besides fs readable streams that are set to 64kb).
Increasing the buffer size allows less allocations to be used in general and increasing the default high water mark reduces the number of chunks which improves the throughout a lot.
I would like to increase the buffer pool size to 128kb. The regular high water mark could increase to 64kb and the high water mark for fs to e.g., 256kb.
Those numbers are all "magical" numbers but they seem better defaults than the current ones. Please let me know if there are reasons to either not change the defaults or if you have an alternative suggestion.
It is also possible to change the Buffer's pool size default during runtime and to set each high water mark per stream.
@nodejs/fs @nodejs/streams @nodejs/buffer PTAL | buffer,fs,stream,performance | low | Major |
430,171,290 | fastapi | ApiKey Header documentation | Hi,
Do you have some documentation or example regarding configuration for ApiKey (header) and how to verify it on FastAPI ?
thanks in advance,
RΓ©my. | good first issue,docs,confirmed,reviewed | high | Critical |
430,179,272 | neovim | Floating windows inherit horizontal scroll offset | - `nvim --version`: v0.4.0-545-gc5e8924f4
- Operating system/version: Mac OS Mojave
- Terminal name/version: kitty 0.13.1
- `$TERM`: xterm-256color
Floating windows apparently inherit the horizontal scroll offset of the currently focused window, if the floating window is not being entered (`nvim_open_win({buffer}, false, {config})`)

I have been able to reproduce this with a _work-in-progress_ [plugin](https://github.com/tbo/notion/blob/master/index.ts#L25) of mine, but I have seen the same behavior with [coc.nvim](https://github.com/neoclide/coc.nvim):
<img width="860" alt="Screenshot 2019-04-07 at 18 48 17" src="https://user-images.githubusercontent.com/264745/55688253-87bb8980-596e-11e9-9d21-01e324cfe091.png">
| bug,needs:decision,needs:discussion,floatwin | low | Major |
430,189,087 | godot | Shader looks different on Android (two devices; GLES2) | <!-- Please search existing issues for potential duplicates before filing yours:
https://github.com/godotengine/godot/issues?q=is%3Aissue
-->
**Godot version:**
master
**OS/device including version:**
Android (zerofltexx Ressurrection OS 9.0; matissewifi Lineage OS 7.1)
**Issue description:**
While the shader looks correct on my smartphone, on my tablet with lineage os there are strange rectangles.
**Steps to reproduce:**
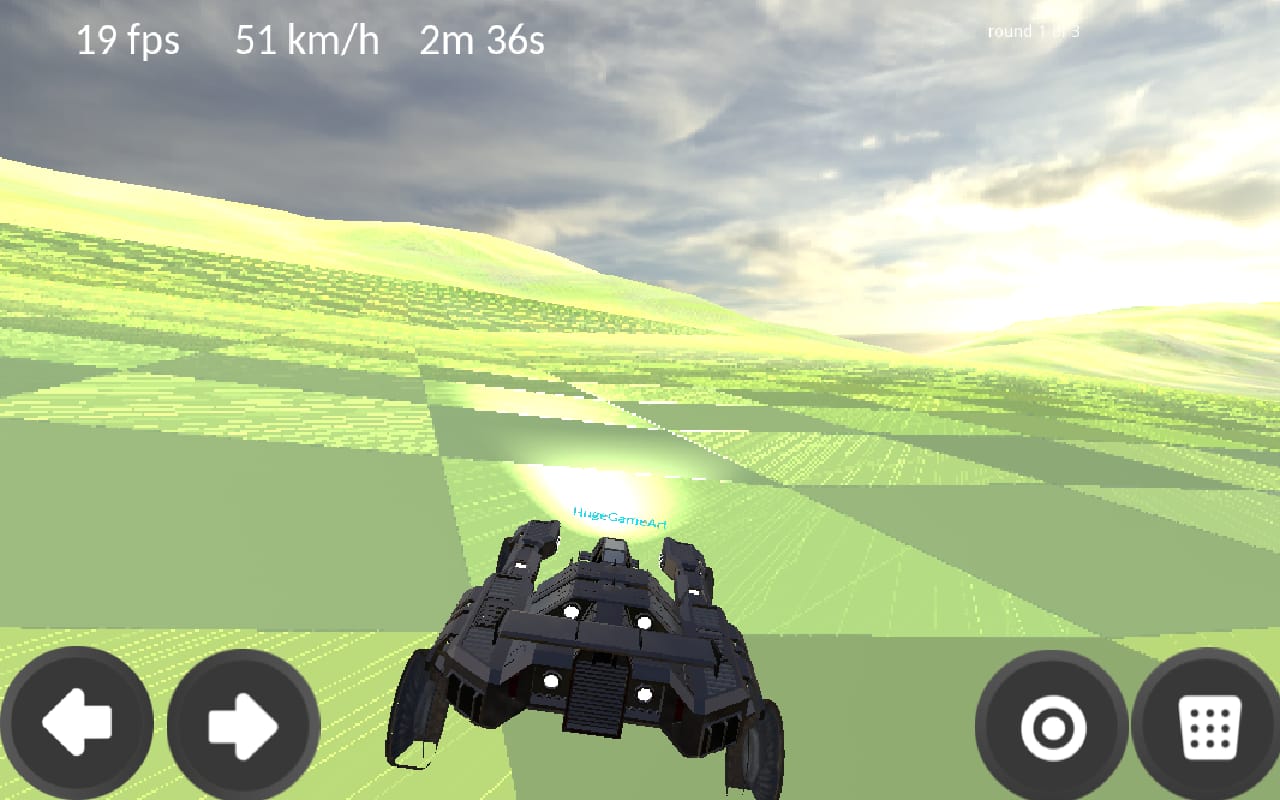
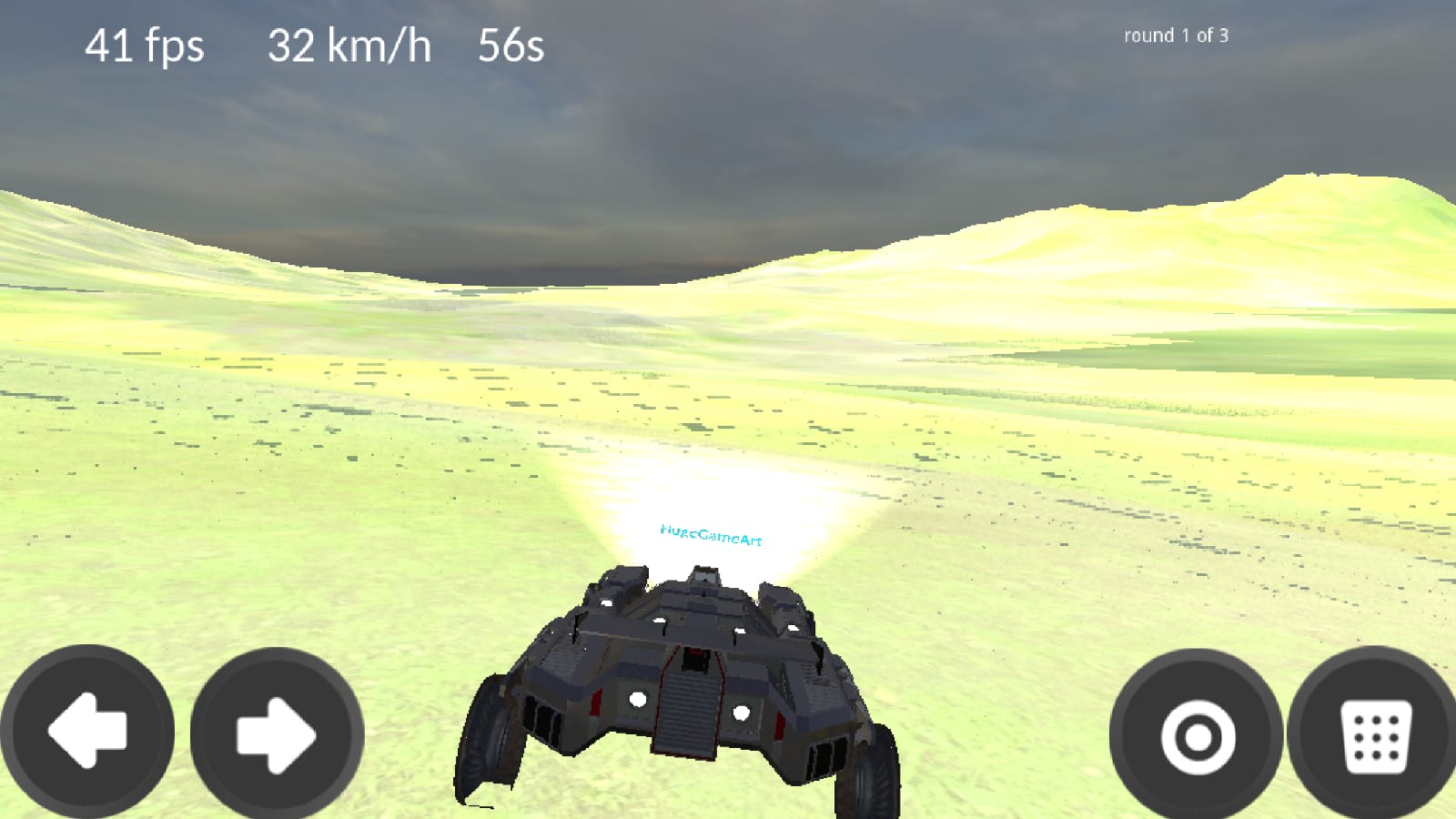
**Minimal reproduction project:**
[Solus-Stunts-v1.0-bug.zip](https://github.com/godotengine/godot/files/6386996/Solus-Stunts-v1.0-bug.zip)
| bug,platform:android,topic:rendering | low | Critical |
430,213,608 | godot | ViewportTexture: Path to node is invalid | ___
***Bugsquad note:** This issue has been confirmed several times already. No need to confirm it further.*
___
**Godot version:** v3.1.stable.mono.official
<!-- Specify commit hash if non-official. -->
**OS/device including version:** Windows 10.0.17763 Build 17763
<!-- Specify GPU model and drivers if graphics-related. -->
**Issue description:**
<!-- What happened, and what was expected. -->
I created a Viewport node and had it draw some GUI. Then, on a MeshInstance (quad) I set its albedo texture to ViewportTexture and selected the viewport node (at Player/Viewport). It still came up with a preview.
When I ran the game I was met with two errors:
```
Node not found: Player/Viewport
ViewportTexture: Path to node is invalid
```
But I was still able to play the game and furthermore, the viewport still worked correctly. I took a look at the scene's `.tscn` file and found that the ViewportTexture does indeed point correctly to the Viewport node I had created.
ViewportTexture, SpatialMaterial, and QuadMesh:
```tscn
...
[sub_resource type="ViewportTexture" id=1]
flags = 1
viewport_path = NodePath("Player/Viewport")
[sub_resource type="SpatialMaterial" id=2]
resource_local_to_scene = true
flags_transparent = true
flags_unshaded = true
flags_albedo_tex_force_srgb = true
albedo_texture = SubResource( 1 )
[sub_resource type="QuadMesh" id=3]
resource_local_to_scene = true
material = SubResource( 2 )
size = Vector2( 0.3, 0.2 )
...
```
**Scene tree:**
```
ββ΄Spatial
β β΄Player
β β β΄Camera
β β β΄Cockpit
β β β΄Right
β β β΄Left
β β β΄CockpitAudio
β β β΄GunFireCockpitAudio
β β β΄TargetingMesh
β ββ΄Viewport
β ββ΄GUI
β ββ΄Speed
β β΄CSGBox
ββ΄CSGBox2
```
This is very similar to #14790, #12781, and #19259.
**Steps to reproduce:**
Create a Viewport node, assign a ViewportTexture to a mesh, select the Viewport you made, and run the game.
| bug,topic:core,confirmed | high | Critical |
430,216,247 | rust | attribute to reserve None value on Option<enum> | For example:
```rust
#[repr(u8)]
#[reserved(0)]
enum Foo {
Bar = 17,
Baz = 32,
}
fn main() {
assert_eq!(std::mem::size_of::<Option<Foo>>(), std::mem::size_of::<Foo>());
let foo: Option<Foo> = None;
let byte: u8 = std::mem::transmute(foo);
println!("{}", byte); // Prints 0
}
```
This would be incredibly useful in FFI situations. Often there is an enum of values for which a particular value (usually zero) is reserved to represent "none" or "invalid" or "empty". This would make translation into idiomatic Rust both easy and safe.
Rust already optimizes `Option<enum>` this way. However, it always selects the highest used discriminant + 1 to represent `None` (this is likely undefined behavior). Having control over this value simply makes the behavior defined. It would also mean that the compiler could warn if there was any attempt to use the reserved value in an actual enumeration item. | A-FFI,T-lang,C-feature-request,needs-rfc,A-enum | low | Minor |
430,217,947 | godot | Socket Error 54 | <!-- Please search existing issues for potential duplicates before filing yours:
https://github.com/godotengine/godot/issues?q=is%3Aissue
-->
**Godot version:**
<!-- Specify commit hash if non-official. -->
3.1.stable.mono.official
**OS/device including version:**
<!-- Specify GPU model and drivers if graphics-related. -->
MacOS Mojave
**Issue description:**
<!-- What happened, and what was expected. -->
I downloaded the procedural planet generation project from:
https://github.com/Bauxitedev/stylized-planet-generator
But when I try to run it, I get the following error:
** Debug Process Started **
drivers/unix/net_socket_posix.cpp:199 - Socket error: 54
** Debug Process Stopped **
**Steps to reproduce:**
Download this github project and try to run.
**Minimal reproduction project:**
<!-- Recommended as it greatly speeds up debugging. Drag and drop a zip archive to upload it. -->
See the github project.
| bug,platform:macos,topic:core | low | Critical |
430,226,483 | flutter | Splash Animation on Icon Button is always behind the Stack | ## Steps to Reproduce
```dart
bottomNavigationBar: Stack(
children: <Widget>[
BottomNavigationBar(
type: BottomNavigationBarType.fixed,
items: [
BottomNavigationBarItem(...),
BottomNavigationBarItem(...),
BottomNavigationBarItem(...),
// Imitate an invisible icon
BottomNavigationBarItem(
icon: const Icon(Icons.add_circle, size: 0),
title: const Text(''),
),
],
currentIndex: _selectedIndex,
fixedColor: Colors.indigo,
onTap: _onItemTapped,
iconSize: 24,
),
Positioned(
right: 32.0,
top: 8.5,
child: CircleAvatar(
child: IconButton(
splashColor: Colors.green,
icon: Icon(Icons.add),
onPressed: () {},
),
),
)
],
),
```
## Description
As you can see the splash for the icon button is positioned behind the bottom navigation.
| Video |
|--------|
| 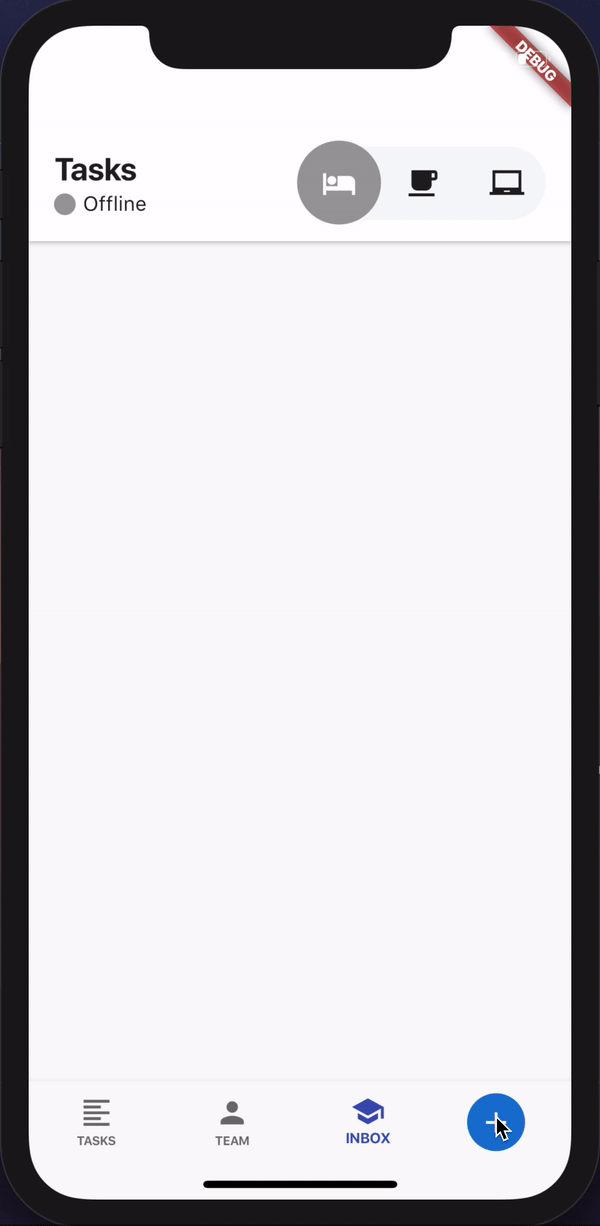 |
| framework,f: material design,a: quality,f: gestures,has reproducible steps,P2,found in release: 3.3,workaround available,found in release: 3.7,team-design,triaged-design | low | Critical |
430,234,651 | go | proposal: net/http: parser for netrc files | There are two implementations of a netrc parser: one lives in src/cmd/go/internal/web2/web.go and the other one lives in x/tools/cmd/auth/netrcauth/netrcauth.go.
Apart from the fact that these two implementations may differ in how they parse the spec this is a useful piece of functionality written by people with a high degree of trust and it would be nice if this library could be imported from somewhere. | Proposal | low | Major |
430,294,890 | godot | Godot crashes when renaming an Audio file | Godot 3.1 stable, Windows 10.
If you attach an Audio file to an AudioStreamPlayer in a scene and then afterwards try to rename the audio file in the the FileSystem then Godot will crash. It does not matter if the scene is open or closed. In our case the audio file was an ogg file.
We also tried testing this with a sprite node but this works as intended, so it might only be a problem with audio files.
To reproduce simply make:
- A new scene and add an AudioStreamPlayer.
- Attach an audio file and save the scene.
- Rename the audio file in the FileSystem
**Edit:** I did some more testing and I found out that this does not happen every time but almost every time. It happens both when the scene is open and closed but it seems to be happen more when it's open.
Edit 2: We also tried on Windows 8.1 but we were only able to reproduce the crash one time after a lot of renaming, so it might be worse on Windows 10 (but it's hard to say). | bug,topic:editor,confirmed,topic:audio,topic:import,crash | low | Critical |
430,400,005 | godot | Option to reorder variables in debug inspector | **Godot version:**
3.2 dev
**OS/device including version:**
The variable inspector for debugging has been quite limited and difficult to handle.
Besides the problem already reported in https://github.com/godotengine/godot/issues/26993, where it is not possible to keep a variable in the same position as the inspector during debugging, also, when there are many variables, they appear in the inspector in an apparently random order, that is, to find a variable in the inspector, one has to make a visual search, variable by variable, until finding the desired variable (and as already said above, all this is lost when debugging with F10 or F12) ...
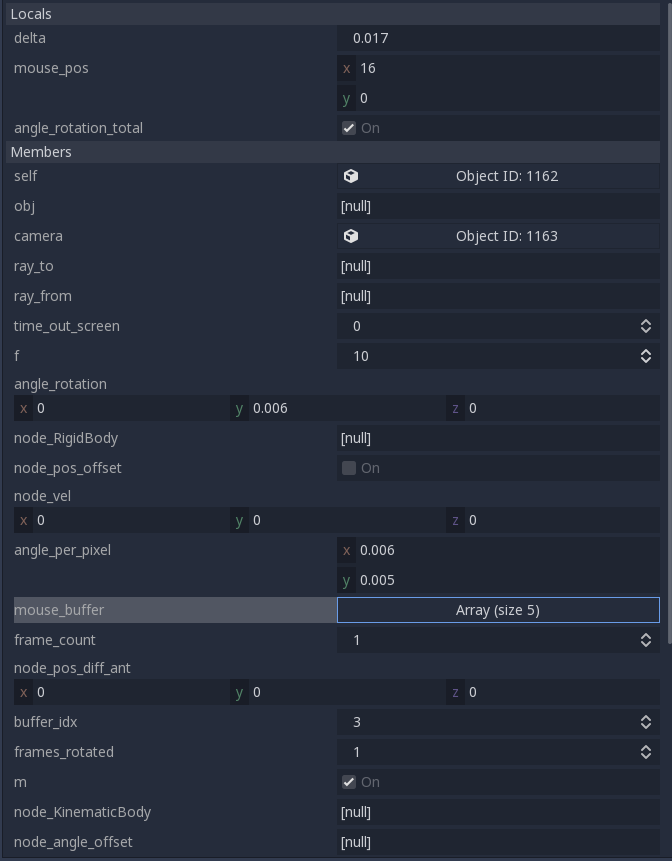
My suggestion:
Allow variables to be **ordered alphabetically by name**, in ascending and descending order.
Also, a **search button** to find a variable name would be pretty cool. | enhancement,topic:editor,usability | low | Critical |
430,400,857 | TypeScript | Suggestion: Type-check statement to verify type assignability | ## Search Terms
type check statement compile (compilation) time
## Suggestion
I wanted to check compatibilities for types from different (especially external) modules in compilation time.
```ts
import { SomeType } from 'some-external';
// ... something statement here to check if SomeType is not changed
// by update for 'some-external' or etc.
```
To solve it, I suggest to add type-check statement like following:
```ts
// Compile error if 'SomeType' does not satisfy the constraint 'WantedType'
// (similar to error TS2344)
type assert SomeType extends WantedType;
// The message "Unexpected 'SomeType'" will be output if the error occurs
// - The trailing expression (message clause) must be a valid string literal
// (the message clause is not necessary for this suggestion
// but would make easier to solve the error...)
type assert SomeType extends WantedType, "Unexpected 'SomeType'";
```
* The type-check process should be same as the process for type constraints in type parameters.
* There is already `type` statement, but the above statements are not conflicted because `type` statements requires `=` after _BindingIdentifier_ (or _TypeParameters_).
* Similar to #10421, but this `type assert` only check types; it does not cast variables.
* `extends` keyword may confuse to one used in conditional type. (not ambiguous?)
* For `type assert A extends B`, both `A` and `B` should accept conditional type.
* (`type assert` is somewhat inspired by other languages' `assert` statements (especially `static_assert` in C/C++), but I don't know whether the words `type assert` are the best...)
## Use Cases
* Check compatibilities for types simply and easily
* Useful to avoid using incompatible package versions with another packages/modules.
* Assert types explicitly with more human-readable
* This would not be useful for compilers/implementers, but would be useful for developers to read codes.
* Test type definition (e.g. for unit test)
Currently we can check types statically as following:
```ts
import { SomeType } from 'some-external';
// Helper definition to check type
type TypeCheckerOfWantedType<T extends WantedType> = T;
// Error if 'SomeType' does not satisfy 'WantedType'
type CheckResultForSomeType = TypeCheckerOfWantedType<SomeType>;
// this is necessary to avoid "'CheckResultForSomeType' is declared but never used"
declare global { var _dummyVariableForCheckSomeType: CheckResultForSomeType; }
```
While no JavaScript codes are generated from this code, this is more complex to check.
## Examples
```ts
// Must not be an error
type assert Element extends Node;
// Error in 'es5', pass in 'es2015'
type assert ObjectConstructor extends { assign: (...args: any[]) => any; },
'Object.assign() is not available';
import { User } from 'db-library';
// Error if User does not have 'id' member with type assignable to 'number'
type assert User extends { id: number; }, 'Unexpected User type';
const a = someFunction();
if (typeof a === 'string') {
// treat 'a' as 'string'
} else {
// Error if narrowed type of 'a' does not have '{ data: string }'
type assert typeof a extends { data: string; };
// (this assert does not mean that type of 'a' is treated as '{ data: string }' here)
}
```
## Checklist
My suggestion meets these guidelines:
* [x] This wouldn't be a breaking change in existing TypeScript/JavaScript code
* [x] This wouldn't change the runtime behavior of existing JavaScript code
* [x] This could be implemented without emitting different JS based on the types of the expressions
* [x] This isn't a runtime feature (e.g. library functionality, non-ECMAScript syntax with JavaScript output, etc.)
* [x] This feature would agree with the rest of [TypeScript's Design Goals](https://github.com/Microsoft/TypeScript/wiki/TypeScript-Design-Goals).
| Suggestion,Awaiting More Feedback | low | Critical |
430,406,772 | rust | Functions with uninhabited return values codegen trap instead of unreachable | With https://github.com/rust-lang/rust/pull/59639, I would expect the following two functions to generate the same LLVM IR:
```rust
#[derive(Clone, Copy)]
pub enum EmptyEnum {}
#[no_mangle]
pub fn empty(x: &EmptyEnum) -> EmptyEnum {
*x
}
#[no_mangle]
pub fn unreach(x: &EmptyEnum) -> EmptyEnum {
unsafe { std::hint::unreachable_unchecked() }
}
```
However, [they do not](https://play.rust-lang.org/?version=nightly&mode=release&edition=2018&gist=ea23cb43f5e0270d834063df075c1495):
```
; playground::empty
; Function Attrs: noreturn nounwind nonlazybind uwtable
define void @_ZN10playground5empty17he3f416ff39576b93E(%EmptyEnum* noalias nocapture nonnull readonly align 1 %x) unnamed_addr #0 {
start:
tail call void @llvm.trap()
unreachable
}
; playground::unreach
; Function Attrs: norecurse noreturn nounwind nonlazybind readnone uwtable
define void @_ZN10playground7unreach17h416ea08edc51ffc9E(%EmptyEnum* noalias nocapture nonnull readonly align 1 %x) unnamed_addr #1 {
start:
unreachable
}
```
Namely, `empty` triggers a well-defined trap while `unreach` causes UB.
That this makes a difference can be seen [when adding](https://play.rust-lang.org/?version=nightly&mode=release&edition=2018&gist=071e2e180cab8170a85fe564d8f86e05):
```rust
pub fn test_unreach(x: bool) -> i32 {
if x {
42
} else {
unsafe { unreach(&*(8 as *const EmptyEnum)) };
13
}
}
pub fn test_empty(x: bool) -> i32 {
if x {
42
} else {
unsafe { empty(&*(8 as *const EmptyEnum)) };
13
}
}
```
The first becomes
```
; playground::test_unreach
; Function Attrs: norecurse nounwind nonlazybind readnone uwtable
define i32 @_ZN10playground12test_unreach17he4cbbc8d69597b0eE(i1 zeroext %x) unnamed_addr #2 {
start:
ret i32 42
}
```
but the second becomes
```
; playground::test_empty
; Function Attrs: nounwind nonlazybind uwtable
define i32 @_ZN10playground10test_empty17hcb53970308f4f532E(i1 zeroext %x) unnamed_addr #3 {
start:
br i1 %x, label %bb1, label %bb2
bb1: ; preds = %start
ret i32 42
bb2: ; preds = %start
tail call void @llvm.trap() #5
unreachable
}
```
because the second branch does not actually cause UB.
Cc @eddyb @cuviper | A-codegen,T-compiler | medium | Major |
430,410,787 | go | cmd/go: version encoding in GOPROXY indexes (list files) | [goproxy](https://tip.golang.org/cmd/go/#hdr-Module_proxy_protocol) states that
> GET $GOPROXY/<module>/@v/list returns a list of all known versions of the given module, one per line.
but also, a few lines later, that
> To avoid problems when serving from case-sensitive file systems, the *module* and *version* elements are case-encoded
That seems to imply that because version is case-encoded in file paths and urls, it is also encoded in lists. However, βseems to implyβ is not the same thing as a clear specification, and
[rewriteVersionList](https://github.com/golang/go/blob/c37b6ecc8ae90fad4c3bde947d96487820cdceec/src/cmd/go/internal/modfetch/cache.go#L503) for example, does not seem to perform any encoding check before writing indexes.
(practically we will need to force lower-casing in the versions we process for other reasons, but the go version spec, unfortunately, is not so strict).
That's one of the many little things that make an official `go mod index` desirable (issue #31303) | NeedsInvestigation,modules | low | Minor |
430,423,925 | three.js | ImageBitmapLoader Cache key should take account of ImageBitmapOptions | ##### Description of the problem
**Background**
`ImageBitmap` is created with `createImageBitmap()`. `createImageBitmap()` can take options `imageOrientation(flipY)`, `premultiplyAlpha`, and so on. The options have effect to bitmap.
https://developer.mozilla.org/en-US/docs/Web/API/WindowOrWorkerGlobalScope/createImageBitmap
So `ImageBitmap`s created with different options parameters should be distinguished from each other even if they refer to the same url.
**Problem**
But `Cache` system in `ImageBitmapLoader` uses only url as cache key.
https://github.com/mrdoob/three.js/blob/dev/src/loaders/ImageBitmapLoader.js#L50
https://github.com/mrdoob/three.js/blob/dev/src/loaders/ImageBitmapLoader.js#L87
In the example below, `bitmap` should be distinguished from `bitmap2` but identical if `Cache` is enabled.
```javascript
var loader = new THREE.ImageBitmapLoader();
loader.setOptions( { imageOrientation: 'none' } );
loader.load( url, function ( bitmap ) {
loader.setOptions( { imageOrientation: 'flipY' } );
loader.load( url, function ( bitmap2 ) {
console.log( bitmap === bitmap2 ); // Should be false
} );
} );
```
##### Three.js version
- [x] Dev
- [ ] r103
- [ ] ...
##### Browser
- [x] All of them
- [ ] Chrome
- [ ] Firefox
- [ ] Internet Explorer
##### OS
- [x] All of them
- [ ] Windows
- [ ] macOS
- [ ] Linux
- [ ] Android
- [ ] iOS
##### Hardware Requirements (graphics card, VR Device, ...)
| Suggestion | low | Minor |
430,429,717 | rust | Request: rustdoc search filter for `unsafe` items | I think that it would be very useful if the pages that rustdoc generated had a way to quickly look at just all of the `unsafe` functions/methods/traits. It would be useful to see both a list of every `unsafe` item and also to have a search be able to "focus" on just `unsafe` items.
| T-rustdoc,C-feature-request,A-type-based-search | low | Minor |
430,490,322 | godot | Editor: context menu for the script symbol | **Godot version:**
3.1 stable
**OS/device including version:**
Win7
**Issue description:**
Right now, the only interaction with the script symbol is LMB click to bring up the script in the script panel.
As editor UI improvement, I request a RMB click to bring up only for this particular script specific options such as
- Remove Script (instead of "clear script" as it is called now in the context menu of the node, which does not actually clear the script, but removes it from the node)
- Remove Script And Delete From res://
- Extend Script
- Copy Script https://github.com/godotengine/godot/issues/27280
- Paste Script (Replace Script) https://github.com/godotengine/godot/issues/27280
- Save Script As ...
- Rename Script
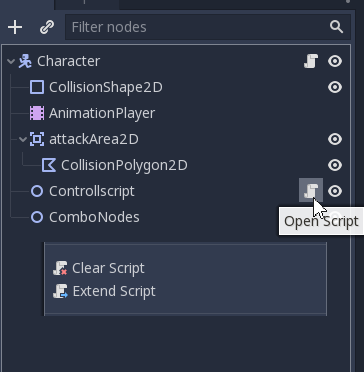
| enhancement,topic:editor,usability | low | Minor |
430,519,480 | pytorch | Clean up and consolidate DDP tests | Tests for `DistributedDataParallel` are scattered in `test_distributed.py` and `test_c10d.py`. There are some duplicated contents, e.g., input modules and skip rules. We should dedup and consolidate existing tests, and extract them into a separate `test_ddp.py` file. | oncall: distributed,module: tests,triaged | low | Minor |
430,547,070 | flutter | Support multiple windows for desktop shells | See discussion in https://github.com/google/flutter-desktop-embedding/issues/98
If we end up supporting multiple root views within one engine instance, this will require embedding API changes. If we don't, we'll need to have a viable strategy to recommend for coordinating between windows that works for cases that require constant synchronization (e.g., a tools palette in one window, with the canvas using those tools in another) | c: new feature,engine,platform-mac,platform-windows,customer: crowd,platform-linux,a: desktop,P3,customer: chalk (g3),a: multi window,team-framework,triaged-framework | high | Critical |
430,551,595 | vue | [template-renderer] Make it possible to not automatically render used async scripts | ### What problem does this feature solve?
In oder to make https://github.com/maoberlehner/vue-lazy-hydration more useful it would be great if we could prevent the template renderer from automatically injecting async scripts. The way vue-lazy-hydration works is, that it behaves differently on the server than it does on the client. On the server the script (of an async component) is loaded immediately so the template renderer correctly detects that it is used. But on the client the script might not be needed at all but because the template renderer has already injected it it is immediately loaded on page load.
There is currently kind of a backlash against loading huge amounts of JavaScript. vue-lazy-hydration can help with removing a lot of unnecessary JavaScript on server side rendered, mostly static sites like blogs and documentation. But currently it can't completely prevent loading all of the unnecessary JavaScript because of the way how template renderer works.
Here is the relevant line in the code: https://github.com/vuejs/vue/blob/dev/src/server/template-renderer/index.js#L226
### What does the proposed API look like?
I propose to make this configurable:
```js
const renderer = createBundleRenderer(serverBundle, {
template,
renderUsedAsyncScripts: false,
});
```
<!-- generated by vue-issues. DO NOT REMOVE --> | feature request | high | Critical |
430,553,658 | go | x/tools/go/packages: fake command-line-args package isn't created on windows when ad-hoc package file is missing | I haven't been able to reproduce this for go list, so I'm setting this as a go/packages bug for now.
It seems like on Windows, when doing a `go list -e` of an ad-hoc package where a source file doesn't exist, the fake `command-line-args` package that contains the error isn't created. See the comment on golang.org/x/tools/go/packages.TestErrorMissingFile. | NeedsInvestigation,Tools | low | Critical |
430,580,917 | flutter | Allow setting desktop shell window title | The closest analog to `SystemChrome.setApplicationSwitcherDescription`'s mobile behavior would be to have it change the title of the window containing the Flutter view. This would definitely be the right behavior for single-window applications, but would also be useful for, e.g., document-style applications where the Flutter code would want to be able to put the document title in the window dynamically based on the content. | c: new feature,engine,platform-mac,platform-windows,platform-linux,c: proposal,a: desktop,e: glfw,P3,team-framework,triaged-framework | medium | Critical |
430,582,110 | pytorch | C++ custom module not thread safe | ## π Bug
<!-- A clear and concise description of what the bug is. -->
If I create multiple threads, each owning its own built in pytorch module such as `torch::Linear`, I don't get thread safety related errors when running the networks concurrently. However, when I implement my own module, I get segmentation faults.
## To Reproduce
I managed to create this MCVE. This does nothing more than
1. define my own convolution block (`ConvBlock`)
2. define a function which will be executed by another thread
3. make the main thread and the subthread perform the exact same operation
Note that the two threads have their own instance of `ConvBlock`. Thus I think it's expected that feed forwarding through these networks separately should be thread safe. However, it's not.
```
#include <iostream>
#include <thread>
#include <torch/torch.h>
struct ConvBlockImpl : torch::nn::Module {
ConvBlockImpl(int in_channel, int C /* channels out */, int K, int P)
: conv(register_module("conv", torch::nn::Conv2d(torch::nn::Conv2dOptions(in_channel, C, K).padding(P)))),
batch_norm(register_module("bn", torch::nn::BatchNorm(C))) { /* pass */ }
torch::Tensor forward(const torch::Tensor& x){
return torch::relu(batch_norm(conv(x)));
}
torch::nn::Conv2d conv;
torch::nn::BatchNorm batch_norm;
};
TORCH_MODULE(ConvBlock);
void foo()
{
std::cout << "Foo: " << std::this_thread::get_id() << std::endl;
ConvBlock net(3, 3, 3, 1);
while (true) {
torch::Tensor x = torch::ones({8,3,3,3});
torch::Tensor out = net->forward(x);
}
}
int main() {
std::cout << "Main: " << std::this_thread::get_id() << std::endl;
std::cout.flush();
std::thread worker(&foo);
ConvBlock net(3, 3, 3, 1);
while (true) {
torch::Tensor x = torch::ones({8,3,3,3});
torch::Tensor out = net->forward(x);
}
worker.join();
return 0;
}
```
Once run (I'm on macOS 10.14.3), the result looks something like
```
Main: 0x119ed55c0
Foo: 0x70000649d000
Segmentation fault: 11
```
<!-- If you have a code sample, error messages, stack traces, please provide it here as well -->
## Expected behavior
<!-- A clear and concise description of what you expected to happen. -->
Two different threads are holding two different instances of my custom made module, thus I assumed that any operations done to each of them shouldn't interfere with the other.
## Environment
- PyTorch Version (e.g., 1.0): libtorch 1.0.1
- OS (e.g., Linux): macOS 10.14.3
- How you installed PyTorch (`conda`, `pip`, source): downloaded libtorch from the website
- Build command you used (if compiling from source):
- Python version: -
- CUDA/cuDNN version: CPU only
- GPU models and configuration: -
- Any other relevant information: - | needs reproduction,module: cpp,triaged | low | Critical |
430,602,928 | flutter | Support Texture widget in GLFW embedding | See google/flutter-desktop-embedding#107 for discussion | c: new feature,engine,c: proposal,a: desktop,e: glfw,P3,team-linux,triaged-linux | low | Major |
430,608,617 | rust | arm-unknown-linux-gnueabi libstd panics at startup with lto enabled | Without lto everything seems fine. | O-Arm,T-compiler,C-bug | low | Minor |
430,627,266 | flutter | Add desktop shell support for text navigation key combinations | On macOS, typing cmd+right invokes moveToRightEndOfLine: These methods are already received by doCommandBySelector: so we we just need to implement the logic.
On glfw we could use modifier flags https://www.glfw.org/docs/latest/group__mods.html#ga14994d3196c290aaa347248e51740274 | c: new feature,engine,platform-mac,platform-windows,platform-linux,c: proposal,a: desktop,e: glfw,P2,team-text-input,triaged-text-input | low | Critical |
430,632,699 | flutter | Add multi-monitor high-DPI support to GLFW shell | The GLFW shell handles monitor resolution, but only based on the primary monitor.
From looking at docs, it seems like `glfwGetWindowContentScale/glfwSetWindowContentScaleCallback` is the right way to handle the multi-monitor case, but that requires GLFW 3.3; 3.2 is still the stable version. Once 3.3 is stable we should look into updating the copy in third_party and implementing this. | c: new feature,engine,c: proposal,a: desktop,e: glfw,P3,team-linux,triaged-linux | low | Major |
430,653,779 | flutter | Support window size/placement on desktop | A Flutter application running on desktop should be able to control its window size and placement. This will require exposing screen information (array of screens with frames in global coordinates) as well to be useful.
This is something that's so basic to a desktop application that I think it makes much more sense to implement as a system channel than a plugin. | c: new feature,framework,engine,platform-mac,platform-windows,customer: crowd,platform-linux,a: desktop,e: glfw,P3,team-framework,triaged-framework | high | Critical |
430,680,948 | go | cmd/go: print useful reason when 'go mod tidy' and 'go get' fail due to missing git authentication credentials | <!-- Please answer these questions before submitting your issue. Thanks! -->
### What version of Go are you using (`go version`)?
<pre>
$ go version
go version devel +cb6646234c Fri Apr 5 20:54:50 2019 +0000 darwin/amd64
</pre>
### Does this issue reproduce with the latest release?
Yes
### What operating system and processor architecture are you using (`go env`)?
<details><summary><code>go env</code> Output</summary><br><pre>
$ go env
GOARCH="amd64"
GOBIN=""
GOCACHE="/Users/Gurpartap/Library/Caches/go-build"
GOEXE=""
GOFLAGS=""
GOHOSTARCH="amd64"
GOHOSTOS="darwin"
GOOS="darwin"
GOPATH="/Users/Gurpartap/Projects/go"
GOPROXY=""
GORACE=""
GOROOT="/Users/Gurpartap/.gimme/versions/go"
GOTMPDIR=""
GOTOOLDIR="/Users/Gurpartap/.gimme/versions/go/pkg/tool/darwin_amd64"
GCCGO="gccgo"
CC="clang"
CXX="clang++"
CGO_ENABLED="1"
GOMOD="/dev/null"
CGO_CFLAGS="-g -O2"
CGO_CPPFLAGS=""
CGO_CXXFLAGS="-g -O2"
CGO_FFLAGS="-g -O2"
CGO_LDFLAGS="-g -O2"
PKG_CONFIG="pkg-config"
GOGCCFLAGS="-fPIC -m64 -pthread -fno-caret-diagnostics -Qunused-arguments -fmessage-length=0 -fdebug-prefix-map=/var/folders/ly/3f450xdn7g3bjzjdkh7y66tm0000gn/T/go-build674837816=/tmp/go-build -gno-record-gcc-switches -fno-common"
</pre></details>
### What did you do?
<!--
If possible, provide a recipe for reproducing the error.
A complete runnable program is good.
A link on play.golang.org is best.
-->
<pre>
$ mkdir fresh && cd fresh # outside $GOPATH
$ set -x -g GO111MODULE on
$ go mod init fresh
$ go get -v -x "google.golang.org/grpc"
</pre>
### What did you expect to see?
#### β’ If user was authenticated to pull from cloud.google.com:
The requested module to be downloaded and defined in go.mod. No problem.
#### β’ If user was not authenticated to pull from cloud.google.com:
This `go get` output needs to convey an actionable error reason for the user to fix the situation. Something like:
<pre>
$ go get "google.golang.org/grpc"
go: finding cloud.google.com/go v0.26.0
go: google.golang.org/[email protected] ->
cloud.google.com/[email protected]: fatal: remote error:
Invalid authentication credentials.
Please generate a new identifier:
https://code.googlesource.com/new-password
</pre>
### What did you see instead?
This `go get` output does not convey any actionable error reason for the user to fix the situation.
<pre>
$ go get "google.golang.org/grpc"
go: finding cloud.google.com/go v0.26.0
go: google.golang.org/[email protected] ->
cloud.google.com/[email protected]: unknown revision v0.26.0
</pre>
I had to dig deeper with `go get -v -x ` to find the command that failed. Thanks @thepudds and @heschik.
Then I had to manually execute the failing command (`git ls-remote -q ...`) to find the actual/useful failure reason.
<details><summary><code>go get -v -x "google.golang.org/grpc"</code> Output</summary><br><pre>
$ go get -v -x "google.golang.org/grpc"
Fetching https://google.golang.org/grpc?go-get=1
Parsing meta tags from https://google.golang.org/grpc?go-get=1 (status code 200)
get "google.golang.org/grpc": found meta tag get.metaImport{Prefix:"google.golang.org/grpc", VCS:"git", RepoRoot:"https://github.com/grpc/grpc-go"} at https://google.golang.org/grpc?go-get=1
mkdir -p /Users/Gurpartap/Projects/go/pkg/mod/cache/vcs # git2 https://github.com/grpc/grpc-go
# lock /Users/Gurpartap/Projects/go/pkg/mod/cache/vcs/53ab5f2f034ba42de32f909aa45670cf730847987f38664c4052b329152ad727.lock# /Users/Gurpartap/Projects/go/pkg/mod/cache/vcs/53ab5f2f034ba42de32f909aa45670cf730847987f38664c4052b329152ad727 for git2 https://github.com/grpc/grpc-go
cd /Users/Gurpartap/Projects/go/pkg/mod/cache/vcs/53ab5f2f034ba42de32f909aa45670cf730847987f38664c4052b329152ad727; git ls-remote -q https://github.com/grpc/grpc-go
6.443s # cd /Users/Gurpartap/Projects/go/pkg/mod/cache/vcs/53ab5f2f034ba42de32f909aa45670cf730847987f38664c4052b329152ad727; git ls-remote -q https://github.com/grpc/grpc-go
Fetching https://cloud.google.com/go?go-get=1
Parsing meta tags from https://cloud.google.com/go?go-get=1 (status code 200)
get "cloud.google.com/go": found meta tag get.metaImport{Prefix:"cloud.google.com/go", VCS:"git", RepoRoot:"https://code.googlesource.com/gocloud"} at https://cloud.google.com/go?go-get=1
mkdir -p /Users/Gurpartap/Projects/go/pkg/mod/cache/vcs # git2 https://code.googlesource.com/gocloud
# lock /Users/Gurpartap/Projects/go/pkg/mod/cache/vcs/b0e27935eb83c1d7843713bafab507e95768b550f0552cb42d9f41e5fd9c8375.lock# /Users/Gurpartap/Projects/go/pkg/mod/cache/vcs/b0e27935eb83c1d7843713bafab507e95768b550f0552cb42d9f41e5fd9c8375 for git2 https://code.googlesource.com/gocloud
go: finding cloud.google.com/go v0.26.0
cd /Users/Gurpartap/Projects/go/pkg/mod/cache/vcs/b0e27935eb83c1d7843713bafab507e95768b550f0552cb42d9f41e5fd9c8375; git tag -l
0.011s # cd /Users/Gurpartap/Projects/go/pkg/mod/cache/vcs/b0e27935eb83c1d7843713bafab507e95768b550f0552cb42d9f41e5fd9c8375; git tag -l
cd /Users/Gurpartap/Projects/go/pkg/mod/cache/vcs/b0e27935eb83c1d7843713bafab507e95768b550f0552cb42d9f41e5fd9c8375; git ls-remote -q https://code.googlesource.com/gocloud
1.439s # cd /Users/Gurpartap/Projects/go/pkg/mod/cache/vcs/b0e27935eb83c1d7843713bafab507e95768b550f0552cb42d9f41e5fd9c8375; git ls-remote -q https://code.googlesource.com/gocloud
go: google.golang.org/[email protected] ->
cloud.google.com/[email protected]: unknown revision v0.26.0
</pre></details>
<pre>
$ git ls-remote -q https://code.googlesource.com/gocloud
fatal: remote error:
Invalid authentication credentials.
Please generate a new identifier:
https://code.googlesource.com/new-password
</pre>
| help wanted,NeedsFix,modules | low | Critical |
430,688,223 | flutter | Not possible to change screen when app inactive | This is somewhere between a 'how do I' and a feature request. What I'm trying to do is essentially block app's screen whenever it isn't the active app (i.e. switcher, when the user pulls down the notification pane on iOS, etc). I've set up a WidgetsBindingObserver to detect when the app switches to Inactive or Paused, and I'm trying to render a different page in that case.
I'm fairly sure this isn't possible within flutter as I've tried using `SchedulerBinding.instance`'s `scheduleForcedFrame` and `scheduleWarmupFrame` which don't work, and they seem like the most reasonable thing to use. With either, the frame isn't actually rendered until the app resumes again.
I don't know if this is actually even possible to do - but it does seem like it is possible with native iOS code. I think `[self.view snapshotViewAfterScreenUpdates:YES];` and possibly `setNeedsDisplay`. I haven't looked into android too much yet.
If anyone has an idea of how this can be done completely within flutter I'd appreciate it, otherwise I'll be looking at doing it natively... | c: new feature,platform-ios,framework,a: fidelity,a: quality,P3,team-ios,triaged-ios | medium | Major |
430,697,545 | flutter | Something is wrong with the Navigator.replace() method. | Hi there,
So the `replace(oldRoute, newRoute)` method on Navigator expects the old route to be already on the history list (asserting that its `_navigator` actually equals to the `this` navigator), but there's a dead lock here:
- When you push a new page, you have to create a route.
- When you want to create a route, you have to create a destination page (widget) in its builder.
As an example:
```dart
final route = PageRouteBuilder(
pageBuilder: (_) => NewPageWidget(),
);
Navigator.of(context).push(route);
```
We are unable to preserve the `route` instance which is transitioning the UI to `NewPageWidget` because the latter is already instantiated. The reason why we need access to the `route` instance is because its `_navigator` property is initialized after it was pushed to the stack.
At some point, we would like to replace the `NewPageWidget` with a another page (it's a requirement, I cannot use `pushReplacement()` or any other method for that matter), but without access to the original `route` instance that originally placed the page on the stack, how exactly can `replace(oldRoute, newRoute)` work?
A deadlock? If not, an example that show cases a possible solution would be more than welcome, thank you! | framework,d: api docs,f: routes,P2,team-framework,triaged-framework | low | Major |
430,700,147 | go | cmd/go: plugin versions do not match when built within vs. outside a module | #### What did you do?
I have simple application that loads Go plugins and allows them to communicate with app via exported interface.
Simplified version can be found [here](https://github.com/zimnx/central).
Shared interface is stored under `ext` directory, real implementation is located under`plugin` directory.
Application passes pointer to real implementation to dynamically loaded plugin which expects interface.
Example plugin code can be found [here](https://github.com/zimnx/plugins).
Unfortunately combination of Go Modules and Go Plugins doesn't work unless go.mod of plugins module has `replace` entry with local relative path for shared interface path.
When I use remote location in plugins module, application crashes because of wrong version of packages.
Build app:
```
~/tmp/go-plugin-bug/central on ξ master β 16:12:19
$ go clean -modcache
~/tmp/go-plugin-bug/central on ξ master β 16:12:21
$ git checkout v1.0.0
Note: checking out 'v1.0.0'.
~/tmp/go-plugin-bug/central on ξ f1d7e9f β 16:12:22
$ go install -a
```
Then build plugin
```
~/tmp/go-plugin-bug/central on ξ f1d7e9f β 16:12:27
$ cd ../plugins
~/tmp/go-plugin-bug/plugins on ξ master β 16:12:28
$ cat go.mod
module github.com/zimnx/plugins
go 1.12
require github.com/zimnx/central v1.0.0
~/tmp/go-plugin-bug/plugins on ξ master β 16:12:30
$ go build -buildmode=plugin -o plugin.so
go: finding github.com/zimnx/central v1.0.0
go: downloading github.com/zimnx/central v1.0.0
go: extracting github.com/zimnx/central v1.0.0
~/tmp/go-plugin-bug/plugins on ξ master β 16:12:39
$ central plugin.so
2019/04/08 16:12:42 cant open plugin: plugin.Open("plugin"): plugin was built with a different version of package github.com/zimnx/central/ext
```
When I change plugins go mod to use local path instead of remote one everything works.
```
~/tmp/go-plugin-bug/plugins on ξ master β 16:12:42
$ cat go.mod
module github.com/zimnx/plugins
go 1.12
require github.com/zimnx/central v1.0.0
replace github.com/zimnx/central => ../central
~/tmp/go-plugin-bug/plugins on ξ master! β 16:14:30
$ go build -buildmode=plugin -o plugin.so
~/tmp/go-plugin-bug/plugins on ξ master! β 16:14:33
$ central plugin.so
hello = world
```
#### What did you expect to see?
Go Modules and plugins working fine when remote path is used.
#### What did you see instead?
Error about different package versions.
#### Does this issue reproduce with the latest release (go1.12.3)?
Yes.
#### System details
```
go version go1.12.3 linux/amd64
GOARCH="amd64"
GOBIN=""
GOCACHE="/home/maciej/.cache/go-build"
GOEXE=""
GOFLAGS=""
GOHOSTARCH="amd64"
GOHOSTOS="linux"
GOOS="linux"
GOPATH="/home/maciej/work"
GOPROXY=""
GORACE=""
GOROOT="/usr/local/go"
GOTMPDIR=""
GOTOOLDIR="/usr/local/go/pkg/tool/linux_amd64"
GCCGO="gccgo"
CC="gcc"
CXX="g++"
CGO_ENABLED="1"
GOMOD="/home/maciej/tmp/go-plugin-bug/plugins/go.mod"
GOROOT/bin/go version: go version go1.12.3 linux/amd64
GOROOT/bin/go tool compile -V: compile version go1.12.3
uname -sr: Linux 4.14.13-041413-generic
Distributor ID: Ubuntu
Description: Ubuntu 17.10
Release: 17.10
Codename: artful
/lib/x86_64-linux-gnu/libc.so.6: GNU C Library (Ubuntu GLIBC 2.26-0ubuntu2.1) stable release version 2.26, by Roland McGrath et al.
gdb --version: GNU gdb (Ubuntu 8.0.1-0ubuntu1) 8.0.1
```
| NeedsInvestigation,modules | medium | Critical |
430,734,103 | godot | Tileset collision points doesn't update properly after modification in editor | Godot version: 3.1-stable
**Issue description:**
Tile collision doesn't update visually until you switch off of it then back when numbers are changed in the editor.
**Steps to reproduce:**
Create TileMap
Add new TileSet
Add image in TileSet editor
New Single Tile
Set Region
Add Rectangle in collision tab
Click collision shape in editor
Edit points by changing the number
Then switch from collision to region back to collision and it will update properly
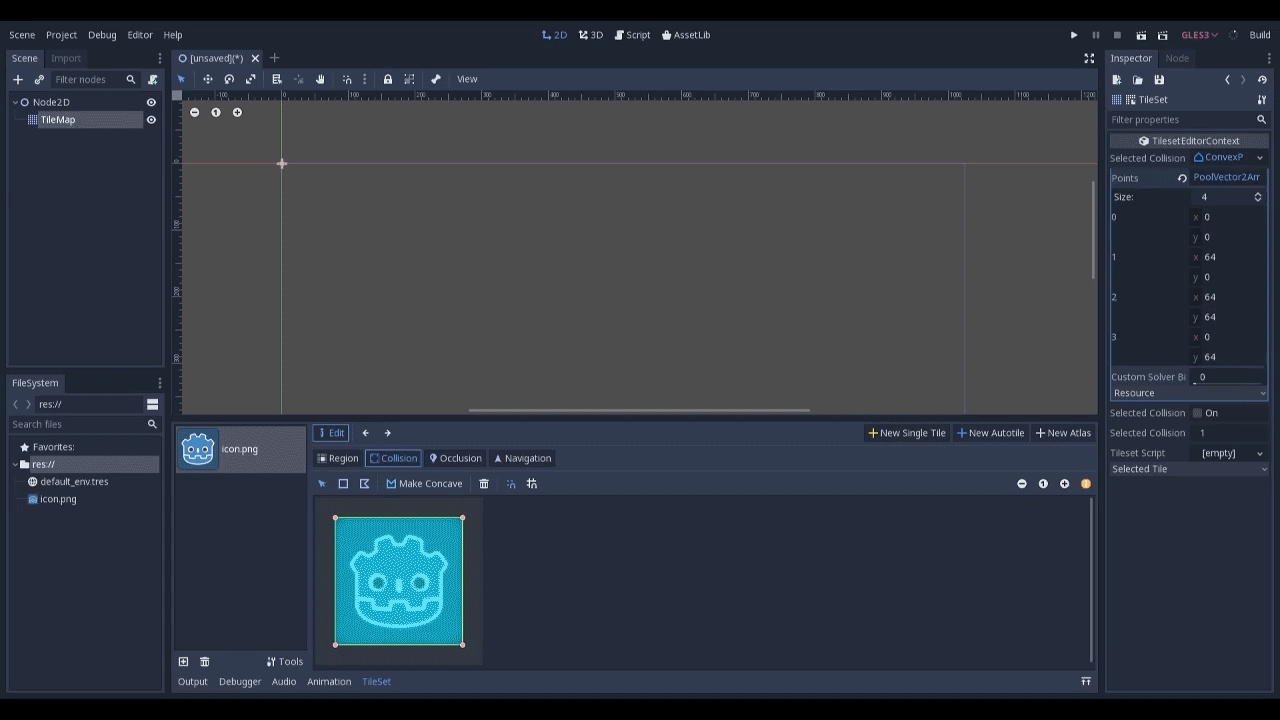
| enhancement,topic:editor,usability | low | Critical |
430,740,717 | pytorch | RNN module weights not compacted | I exported a JIT model and load it in C++, it runs well on CPU. but when I run it on GPU, I get this warning and then the program crashed.
``` text
Warning: RNN module weights are not part of single contiguous chunk of memory.
This means they need to be compacted at every call, possibly greatly increasing memory usage.
To compact weights again call flatten_parameters(). (_cudnn_impl at ..\aten\src\ATen\native\cudnn\RNN.cpp:1249)
```
I tried vgg16 net module before, and it worked well both on CPU and GPU.
I tried to read the API documents, but I can't find flatten_parameters() function. And there is no usage document for other APIs.
Here is my code
```cpp
std::shared_ptr<torch::jit::script::Module> module = torch::jit::load(
"E:/temp/stm.pt", c10::kCUDA);
assert(module != nullptr);
cout << "load module ok!" << endl;
//module->to(at::kCPU);
module->to(at::kCUDA);
cv::Mat img;
cv::Mat img_float;
img = cv::imread("E:/pics/00.jpg", IMREAD_GRAYSCALE);
//cv::cvtColor(img, img, cv::COLOR_BGR2GRAY);
img.convertTo(img_float, CV_32F, 1.0 / 255.0);
cv::resize(img_float, img_float, cv::Size(160, 32));
auto img_tensor = torch::CPU(torch::kFloat32).tensorFromBlob(img_float.data, { 1, 32, 160, 1 });
img_tensor = img_tensor.permute({ 0, 3, 1, 2 });
img_tensor[0][0] = img_tensor[0][0].sub_(0.5).div_(0.5);
auto img_var = torch::autograd::make_variable(img_tensor, false);
cout << img_var.sizes() << endl;
vector<torch::jit::IValue> inputs;
//inputs.push_back(img_var.to(at::kCPU));
inputs.push_back(img_var.to(at::kCUDA));
auto output = module->forward(inputs).toTensor();
auto res = output.max(2);
auto predicts = get<1>(res);
cout << predicts;
```
And [this link](https://github.com/Sierkinhane/crnn_chinese_characters_rec/tree/master/trained_models) is the pytorch model I use.
cc @suo | oncall: jit | low | Critical |
430,784,518 | flutter | [Plugin]Camera plugin does't contain GPS info | ## Use https://github.com/flutter/plugins/tree/master/packages/camera
Take photos
## The photo does not contain GPS info,but if I use phone photo App itself take photos contain GPS info
```
[β] Flutter (Channel stable, v1.2.1, on Mac OS X 10.13.6 17G6030, locale en-CN)
β’ Flutter version 1.2.1 at /Users/tam/Work/TOOLS/flutter
β’ Framework revision 8661d8aecd (8 weeks ago), 2019-02-14 19:19:53 -0800
β’ Engine revision 3757390fa4
β’ Dart version 2.1.2 (build 2.1.2-dev.0.0 0a7dcf17eb)
[β] Android toolchain - develop for Android devices (Android SDK version 28.0.3)
β’ Android SDK at /Users/tam/Library/Android/sdk
β’ Android NDK location not configured (optional; useful for native profiling support)
β’ Platform android-28, build-tools 28.0.3
β’ ANDROID_HOME = /Users/tam/Library/Android/sdk
β’ Java binary at: /Applications/Android Studio.app/Contents/jre/jdk/Contents/Home/bin/java
β’ Java version OpenJDK Runtime Environment (build 1.8.0_152-release-1248-b01)
β’ All Android licenses accepted.
[β] iOS toolchain - develop for iOS devices (Xcode 10.1)
β’ Xcode at /Applications/Xcode.app/Contents/Developer
β’ Xcode 10.1, Build version 10B61
β’ ios-deploy 1.9.4
β’ CocoaPods version 1.5.3
[β] Android Studio (version 3.3)
β’ Android Studio at /Applications/Android Studio.app/Contents
β’ Flutter plugin version 33.3.1
β’ Dart plugin version 182.5215
β’ Java version OpenJDK Runtime Environment (build 1.8.0_152-release-1248-b01)
[β] VS Code (version 1.32.3)
β’ VS Code at /Applications/Visual Studio Code.app/Contents
β’ Flutter extension version 2.25.0
``` | c: new feature,p: camera,package,team-ecosystem,P3,triaged-ecosystem | low | Minor |
430,797,911 | godot | Textures bleeding white when using an UV-based texture atlas with mipmapping | <!-- Please search existing issues for potential duplicates before filing yours:
https://github.com/godotengine/godot/issues?q=is%3Aissue
-->
**Godot version:** 3.1 64b
<!-- Specify commit hash if non-official. -->
**OS/device including version:** Linux 64b, GTX 1070 (proprietary drivers v418)
<!-- Specify GPU model and drivers if graphics-related. -->
**Issue description:**
<!-- What happened, and what was expected. -->
Here is an example: 
All those white tops on green blocks are supposed to be green, it is the same green texture.
I have half of texture size padding around block textures (all block textures are combined in one texture).
What I tried:
* Enabling mipmap on texture didn't change anything.
* Enabling "Use Nearest Mipmap Filter" didn't help.
* "Use Pixel Snap" didn't help (I believe it only works for 2D).
* "Foce Vertex Shading"
* Disabling HDR
* Same thing happens on GLES2 (pic is from GLES3).
* Changing "Anisotropic Filter Level" from 4 to 1 and 8 had no effect.
* Disabling SSAO
* Disabling shadows (in directional light) and light itself
* Disabling reflections
* Disabling depth pass didn't help.
* Changing roughness and albedo didn't help.
* Removing alpha channel from texture didn't help.
**Steps to reproduce:**
Place a box with UV mapping limiting its texture just to a small part, increase camera visibility range, move block far away.
**Minimal reproduction project:**
<!-- Recommended as it greatly speeds up debugging. Drag and drop a zip archive to upload it. -->
[bleeding_white.zip](https://github.com/godotengine/godot/files/3057483/bleeding_white.zip)
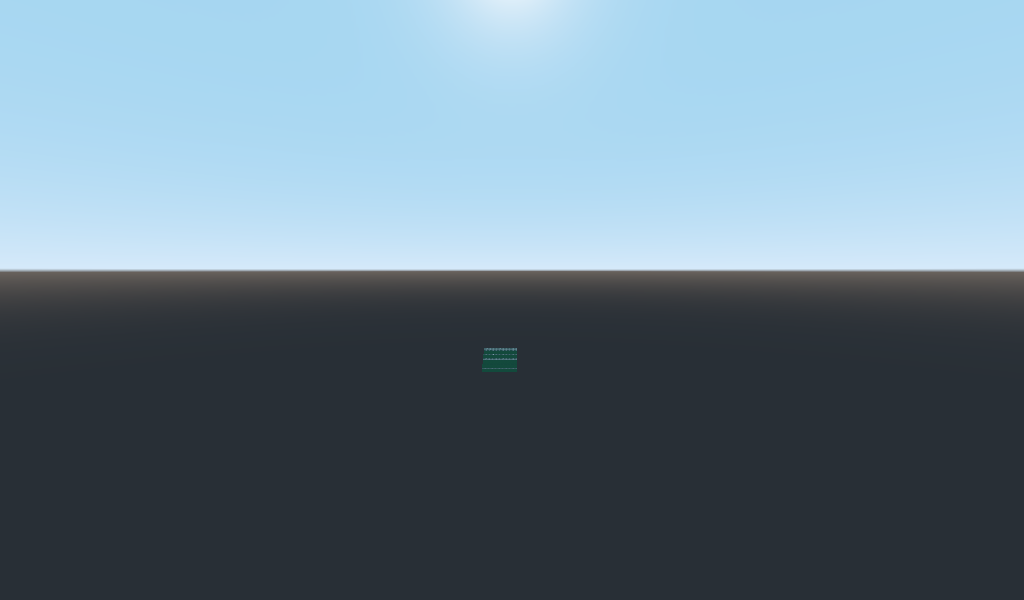
| bug,topic:rendering,confirmed,topic:3d | medium | Critical |
430,839,900 | bitcoin | macOS App Notarization & Stapling | > The Apple notary service is an automated system that scans your software for malicious content, checks for code-signing issues, and returns the results to you quickly. If there are no issues, the notary service generates a ticket for you to staple to your software; the notary service also publishes that ticket online where Gatekeeper can find it.
> Beginning in **macOS 10.14.5**, all new or updated kernel extensions and all software from developers new to distributing with Developer ID must be notarized in order to run. In a future version of macOS, notarization will be required by default for all software.
The currently available version of macOS is 10.14.4.
Apple's notary service requires you to adopt the following protections:
* Enable code-signing for all of the executables you distribute.
* Enable the Hardened Runtime capability for your executable targets, as described in Enable hardened runtime.
* Use a `Developer ID` application, kernel extension, or installer certificate for your code-signing signature.
* Include a secure timestamp with your code-signing signature. (The Xcode distribution workflow includes a secure timestamp by default. For custom workflows, include the `--timestamp` option when running the codesign tool.)
* Donβt include the `com.apple.security.get-task-allow` entitlement with the value set to any variation of true.
* Link against the macOS 10.9 or later SDK.
More info available here: https://developer.apple.com/documentation/security/notarizing_your_app_before_distribution | macOS,Build system | high | Critical |
430,896,609 | go | math: remove assembly stubs | I could be wrong, but I think now that we have mid-stack inlining it should be possible to move the math package from this pattern:
```go
// F does blah.
func F() // implemented in asm, might just jump straight to f
func f() {
// generic implementation in Go
}
```
To something more like this pattern:
```go
// F does blah.
func F() {
f() // might call asm, might just call fGeneric
}
func fGeneric() {
// generic implementation in Go
}
```
This would have the side benefit of allowing architectures that use the generic implementation to inline it if it is cheap enough. It might also make stdlib easier to build with other compilers (#31330). | help wanted,NeedsInvestigation | low | Minor |
430,969,309 | angular | Enable all Angular Bazel Tests on Windows | # π bug report
### Is this a regression?
Nope
### Description
Angular tests are only partially enabled on Windows:
https://github.com/angular/angular/blob/1102b02406ca9fac7cfc7059bc61b4d40d3dd378/.codefresh/codefresh.yml#L22-L24
To have better Windows support, we should be able to run all tests on Windows, that is:
```
bazel test //... --build_tag_filters=-ivy-only --test_tag_filters=-ivy-only
yarn test-ivy-aot //...
```
(IIUC, `yarn test-ivy-aot //...` is essentially `bazel test --define=compile=aot --build_tag_filters=-no-ivy-aot,-fixme-ivy-aot --test_tag_filters=-no-ivy-aot,-fixme-ivy-aot //...`)
I tried to run all tests without `ivy-only` with Bazel 0.24.1 and got
```
Executed 102 out of 175 tests: 86 tests pass, 72 fail to build and 17 fail locally.
```
See full detail at [Angular test with Bazel 0.24.1](https://gist.github.com/meteorcloudy/e1d88b35ff1075e5e9c18f5a80f9aedd)
It turned out all broken tests are because `powershell.exe` is not available when using `--incompatible_strict_action_env`. So I sent a change in Bazel to fix this problem. https://github.com/bazelbuild/bazel/commit/6e752195f0b0c6d67822368b82c990dff168a258
With Bazel@6e75219, after working around some incompatibility issues, I'm able to build and run all Angular tests on Windows. The results are:
- [Angular tests with Bazel@6e75219, excluding `ivy-only`](https://gist.github.com/meteorcloudy/a213a349e88095ef7f9dfe96e1561393)
```
Executed 175 out of 175 tests: 152 tests pass and 23 fail locally.
```
- [Angular tests with Bazel@6e75219, `ivy-only`](https://gist.github.com/meteorcloudy/97d57d4aefd03988efbc1efdf1d0fa09)
```
Executed 7 out of 147 tests: 140 tests pass and 7 fail locally.
```
Current TODO list is:
- [ ] Wait for a Bazel release that contains https://github.com/bazelbuild/bazel/commit/6e752195f0b0c6d67822368b82c990dff168a258, should be 0.26 (Do you prefer this to be cherry-pick into 0.25, so that we can have it this month?)
- [ ] Fix incompatibility issues:
1. https://github.com/angular/angular/pull/29777
2. `bazel-0.24.1 build --nobuild //... --incompatible_bzl_disallow_load_after_statement`
3. Wait for a fix (https://github.com/google/brotli/pull/748) for brotli, then update org_brotli
- [ ] Look into failing tests with Bazel@6e75219, will provide a way to reproduce.
## π¬ Minimal Reproduction
```
# Get Bazel binary built at 6e752195f0b0c6d67822368b82c990dff168a258
wget https://storage.googleapis.com/bazel-builds/artifacts/windows/6e752195f0b0c6d67822368b82c990dff168a258/bazel -O <your preferred Bazel path (with .exe extension)>
# Cherry-pick https://github.com/angular/angular/pull/29777 if it's still not merged.
# Run tests except ivy-only
bazel test //... --build_tag_filters=-ivy-only --test_tag_filters=-ivy-only --incompatible_bzl_disallow_load_after_statement=false
# Run ivy-only tests
bazel test //... --define=compile=aot --build_tag_filters=-no-ivy-aot,-fixme-ivy-aot --test_tag_filters=-no-ivy-aot,-fixme-ivy-aot --incompatible_bzl_disallow_load_after_statement=false
```
Note that we still need `--incompatible_bzl_disallow_load_after_statement=false` until the incompatibility issue is fixed.
If you failed to build brotli, you can use the following in `packages/bazel/package.bzl`:
```
http_archive(
name = "org_brotli",
sha256 = "44ebae3308fd1a757fdc4891e1e1e61ab964afb223ba298d2ea0c29a49564f53",
strip_prefix = "brotli-7388ebb113f0e1dbf4ab0f2daddf036abf29b8e6",
url = "https://github.com/meteorcloudy/brotli/archive/7388ebb113f0e1dbf4ab0f2daddf036abf29b8e6.zip",
)
```
## π Your Environment
**Angular Version:**
<pre><code>
ec5635430630fb895ddb43017f9939564824e8f8
</code></pre>
@alexeagle @filipesilva Can you point this issue to correct people in Angular Windows team? I will also continue to help investigating. | type: bug/fix,area: build & ci,freq2: medium,area: bazel,windows,P3 | low | Critical |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.