id
int64 393k
2.82B
| repo
stringclasses 68
values | title
stringlengths 1
936
| body
stringlengths 0
256k
โ | labels
stringlengths 2
508
| priority
stringclasses 3
values | severity
stringclasses 3
values |
---|---|---|---|---|---|---|
395,471,440 | flutter | iOS Google Maps: Strange appear animation | ## Issue
An issue with Flutter Google maps plugin on iOS both in debug and release builds which occurs when you open an app from a terminated state. No issue on Android
There's a weird appear animation playing when I have Google Map in my widget. A video showcasing the issue https://www.youtube.com/watch?v=94ee6cePbWw&feature=youtu.be
If you play it frame by frame, you'll see that app bar is colored correctly, floating action button is drawn, but then everything is flashed white and floating action button suddenly plays a slide in appearing animation, when it was already displayed. It all looks very glitchy
If I remove Google map from my widget, everything works fine: there's no FAB slide in animation and app bar is not flashed white, after they were drawn.
P.S. Using v0.0.3+3 Google Maps plugin
## Code
```dart
import 'package:flutter/material.dart';
import 'package:google_maps_flutter/google_maps_flutter.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: '',
theme: ThemeData(primarySwatch: Colors.blue),
home: MyHomePage(title: ''),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key, this.title}) : super(key: key);
final String title;
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('')),
body: GoogleMap(
onMapCreated: (controller) {},
),
floatingActionButton: FloatingActionButton(
onPressed: () {},
),
floatingActionButtonLocation: FloatingActionButtonLocation.centerFloat,
);
}
}
```
```
Doctor summary (to see all details, run flutter doctor -v):
[โ] Flutter (Channel dev, v1.1.5, on Mac OS X 10.14.1 18B75, locale en-UA)
[โ] Android toolchain - develop for Android devices (Android SDK version 27.0.3)
[!] iOS toolchain - develop for iOS devices (Xcode 10.1)
โ Verify that all connected devices have been paired with this computer in
Xcode.
If all devices have been paired, libimobiledevice and ideviceinstaller may
require updating.
To update with Brew, run:
brew update
brew uninstall --ignore-dependencies libimobiledevice
brew uninstall --ignore-dependencies usbmuxd
brew install --HEAD usbmuxd
brew unlink usbmuxd
brew link usbmuxd
brew install --HEAD libimobiledevice
brew install ideviceinstaller
[โ] Android Studio (version 3.2)
[!] IntelliJ IDEA Ultimate Edition (version 2018.2.5)
โ Flutter plugin not installed; this adds Flutter specific functionality.
โ Dart plugin not installed; this adds Dart specific functionality.
[โ] Connected device (1 available)
```
| platform-ios,a: quality,customer: crowd,p: maps,package,has reproducible steps,P2,found in release: 2.0,team-ios,triaged-ios | low | Critical |
395,474,101 | godot | Image.resize() biases first row+column in all interpolation modes | **Godot version:**
3.0.6
**Issue description:**
See video.
https://cdn.discordapp.com/attachments/477544613511692358/530275849467985920/2019-01-03_00-46-04.mp4
When trying to help someone with a problem they were having with creating an "average" of a few pixels from a viewport texture (by resizing the raw image data to 1x4), they noticed that the output values were not what they expected. I wrote a test program to see if I could understand what was going on and noticed that as an image is sized down, there appears to be an increasing bias on the leading edge pixels towards what the averaged output would be. This seems to apply regardless of the interpolation method used.
It should be noted that even in "uneven" sized images (not power of 2) this also seems to happen. I tested this with an image with a small amount of alpha 0 in the corner. Eventually, the leading edge on both axes continues to approach a total bias on the interpolated average as the final image size approaches 1 pixel. In the case of the test with the 0-alpha pixel at the first row and column, this meant that an image resized to 2x2 would have 3/4 of its area blank.
Attached is the demo project.
**Minimal reproduction project:**
[MinimalResizeBug.zip](https://github.com/godotengine/godot/files/2722912/MinimalResizeBug.zip)
| bug,topic:rendering,confirmed | low | Critical |
395,474,747 | opencv | python cv2.projectPoints Assertion failed | <!--
If you have a question rather than reporting a bug please go to http://answers.opencv.org where you get much faster responses.
If you need further assistance please read [How To Contribute](https://github.com/opencv/opencv/wiki/How_to_contribute).
Please:
* Read the documentation to test with the latest developer build.
* Check if other person has already created the same issue to avoid duplicates. You can comment on it if there already is an issue.
* Try to be as detailed as possible in your report.
* Report only one problem per created issue.
This is a template helping you to create an issue which can be processed as quickly as possible. This is the bug reporting section for the OpenCV library.
-->
##### System information (version)
<!-- Example
- OpenCV => 3.1
- Operating System / Platform => Windows 64 Bit
- Compiler => Visual Studio 2015
-->
- python => 3.6.5
- OpenCV => 3.4.5
- Operating System / Platform => windows 10
##### Detailed description
cv2.projectPoints produced different results with the same numpy array. One shows "assertion failed" while the other not. Is this a bug?
##### Steps to reproduce
<!-- to add code example fence it with triple backticks and optional file extension
```.cpp
// C++ code example
```
or attach as .txt or .zip file
-->
```
import numpy as np
import cv2
rvec = np.array([0.,0.,0.])
tvec = np.array([0.,0.,0.])
cameraMatrix = np.eye(3)
distCoeffs = np.array([0.,0.,0.,0.])
point = np.zeros([5,4,1])[:,:3,0]
points2d, _ = cv2.projectPoints(point, rvec, tvec, cameraMatrix, distCoeffs)
# ...\opencv-python\opencv\modules\calib3d\src\calibration.cpp:3310: error: (-215:Assertion failed) npoints >= 0 && (depth == CV_32F || depth == CV_64F) in function 'cv::projectPoints'
point1 = point.copy()
points2d, _ = cv2.projectPoints(point1, rvec, tvec, cameraMatrix, distCoeffs)
# OK
point2 = np.zeros([5,3,1])[:,:3,0]
points2d, _ = cv2.projectPoints(point2, rvec, tvec, cameraMatrix, distCoeffs)
# Also OK
# point, point1, point2 has same shape and dtype
```
| category: python bindings | low | Critical |
395,493,005 | rust | Rust stable, fatal runtime error: stack overflow, PartialEq | # Error
```
cargo run
Compiling stack_overflow v0.1.0 (/d/stack_overflow)
Finished dev [unoptimized + debuginfo] target(s) in 7.08s
Running `target/debug/stack_overflow`
thread 'main' has overflowed its stack
fatal runtime error: stack overflow
ะะฒะฐัะธะนะฝัะน ะพััะฐะฝะพะฒ (ััะตะบ ะฟะฐะผััะธ ัะฑัะพัะตะฝ ะฝะฐ ะดะธัะบ)
```
# Rust Version
```
RUST STABLE:
rustc 1.31.1 (b6c32da9b 2018-12-18)
RUST NIGHTLY:
rustc 1.33.0-nightly (9eac38634 2018-12-31)
```
# Code
```
use std::fmt::Write;
use std::ops::Deref;
use std::fmt;
//////The structure stores in itself the changeable reference and has to clean data in "drop" of structure.
#[derive(Debug)]
pub struct DebugSliceMutString<'a>(&'a mut String);
//Stack overflow <----
//assert_eq!(&null.my_debug(&mut string).unwrap(), "=");
impl<'a> PartialEq<str> for DebugSliceMutString<'a> {
fn eq(&self, other: &str) -> bool {
self == other
}
}
//Stack overflow <----
//assert_eq!(null.my_debug(&mut string).unwrap(), "=");
impl<'a> PartialEq<&str> for DebugSliceMutString<'a> {
fn eq(&self, other: &&str) -> bool {
self == other
}
}
//If to take 'deref' that there is no overflow of a stack!!
//assert_eq!(*null.my_debug(&mut string).unwrap(), "=");
impl<'a> Deref for DebugSliceMutString<'a> {
type Target = String;
fn deref(&self) -> &String {
self.0
}
}
impl<'a> Drop for DebugSliceMutString<'a> {
fn drop(&mut self) {
self.0.clear()
}
}
//MyTrait
pub trait MyDebug {
fn my_debug<'a>(&self, w: &'a mut String) -> Result<DebugSliceMutString<'a>, fmt::Error>;
}
impl MyDebug for (usize, usize) {
fn my_debug<'a>(&self, w: &'a mut String) -> Result<DebugSliceMutString<'a>, fmt::Error> {
write!(w, "{}={}", self.0, self.1)?;
Ok( DebugSliceMutString(w) )
}
}
//
fn main() {
//The buffer for an exception of frequent reallocations of memory
let mut string = String::with_capacity(9);
//Any other type, in this case (usize, usize) is more convenient
let null = (10, 20);
// !!!!!!!
//thread 'main' has overflowed its stack
//fatal runtime error: stack overflow
//ะะฒะฐัะธะนะฝัะน ะพััะฐะฝะพะฒ (ััะตะบ ะฟะฐะผััะธ ัะฑัะพัะตะฝ ะฝะฐ ะดะธัะบ)
assert_eq!( null.my_debug(&mut string).unwrap(), "=");
// !!!!!!!
//If to use Deref that there is no overflow of a stack!!
//assert_eq!( *null.my_debug(&mut string).unwrap(), "=");
// !!!!!!!OR
//thread 'main' has overflowed its stack
//fatal runtime error: stack overflow
//ะะฒะฐัะธะนะฝัะน ะพััะฐะฝะพะฒ (ััะตะบ ะฟะฐะผััะธ ัะฑัะพัะตะฝ ะฝะฐ ะดะธัะบ)
//
//let a = null.my_debug(&mut string).unwrap()
// .eq(&"=");
//
//
println!("string, {}, capacity: {}", string, string.capacity());
}
```
# Run
https://play.rust-lang.org/?version=stable&mode=debug&edition=2018&gist=5bacc9a73f0ae7a38100da9d196b08d0
| A-lints,T-compiler,C-feature-request | low | Critical |
395,503,228 | go | plugin: can't open the same plugin with different names, like dlopen | <!-- Please answer these questions before submitting your issue. Thanks! -->
### What version of Go are you using (`go version`)?
<pre>
$ go version
go version go1.11.4 linux/386
</pre>
### Does this issue reproduce with the latest release?
### What operating system and processor architecture are you using (`go env`)?
<details><summary><code>go env</code> Output</summary><br><pre>
$ go env
GOARCH="386"
GOBIN=""
GOCACHE="/home/pw/.cache/go-build"
GOEXE=""
GOFLAGS=""
GOHOSTARCH="386"
GOHOSTOS="linux"
GOOS="linux"
GOPATH="/home/pw/go"
GOPROXY=""
GORACE=""
GOROOT="/usr/local/go"
GOTMPDIR=""
GOTOOLDIR="/usr/local/go/pkg/tool/linux_386"
GCCGO="gccgo"
GO386="sse2"
CC="gcc"
CXX="g++"
CGO_ENABLED="1"
GOMOD="/home/pw/Documents/hello/go.mod"
CGO_CFLAGS="-g -O2"
CGO_CPPFLAGS=""
CGO_CXXFLAGS="-g -O2"
CGO_FFLAGS="-g -O2"
CGO_LDFLAGS="-g -O2"
PKG_CONFIG="pkg-config"
GOGCCFLAGS="-fPIC -m32 -pthread -fmessage-length=0 -fdebug-prefix-map=/tmp/go-build808633565=/tmp/go-build -gno-record-gcc-switches"
</pre></details>
### What did you do?
```
go build -buildmode=plugin -o scanner.so scanner.go
cp scanner.so scanner2.so
```
``` go
package main
import (
"fmt"
"os"
"plugin"
)
type Greeter interface {
Greet()
}
func main() {
plug, err := plugin.Open("./plugins/scanner.so")
if err != nil {
fmt.Println(err)
os.Exit(1)
}
symGreeter, err := plug.Lookup("Greeter")
if err != nil {
fmt.Println(err)
os.Exit(1)
}
var greeter Greeter
greeter, ok := symGreeter.(Greeter)
if !ok {
fmt.Println("unexpected type from module symbol")
os.Exit(1)
}
plug2, err := plugin.Open("./plugins/scanner2.so")
if err != nil {
fmt.Println(err)
os.Exit(1)
}
symGreeter2, err := plug2.Lookup("Greeter")
if err != nil {
fmt.Println(err)
os.Exit(1)
}
var greeter2 Greeter
greeter2, ok2 := symGreeter2.(Greeter)
if !ok2 {
fmt.Println("unexpected type from module symbol")
os.Exit(1)
}
// 4. use the module
greeter.Greet()
greeter2.Greet()
}
```
<!--
If possible, provide a recipe for reproducing the error.
A complete runnable program is good.
A link on play.golang.org is best.
-->
### What did you expect to see?
Same plugin copyed to different names can plugin.Open successfully and have different instances.
For example, I have a lot of scanners connect to my device and the number of scanners is uncertain,
the scanners have same protocol but the serial port is different, so I need to load the same scanner plugin and pass different serial port to the plugin to scan,so it is not suitable to complie different plugin by 'pluginpath', if I need 100 scanners I need to complie 100 times. Why can't just like `dlopen` distinguish by names?
### What did you see instead?
```
plugin.Open("./plugins/scanner2.so"): plugin already loaded
```
| NeedsInvestigation,compiler/runtime | medium | Critical |
395,540,812 | go | cmd/go: when testing a package, also build the non-test variant | go version devel +0175064e69 Thu Jan 3 05:07:58 2019 +0000 linux/amd64
If a package can be built only in test mode, `go test` succeeds while `go build/install` fails:
```
// broken.go
package broken
var X typ
```
```
// broken_test.go
package broken
import "testing"
type typ int
func TestX(t *testing.T) {
var _ typ = X
}
```
If one develops just a package, `go test` is the main means of ensuring that everything works and lots of CIs just run `go test`, which means that it's possible to commit code that does not even build without noticing it.
It would be nice if `go test` would ensure that a package actually builds. | NeedsInvestigation,FeatureRequest,GoCommand | low | Critical |
395,552,584 | angular | Angular animation - nested animation inside *ngIf performance problem | # ๐ bug report
### Affected Package
@angular/animations
### Description
Suppose we have this simple animation:
```
trigger('fadeOut', [
transition(':leave', [
animate(
'0.2s',
style({
height: 0,
opacity: 0
})
)
])
])
```
and this html structure:
```
<div *ngFor="let item of items" [@fadeOut]> <!--- 10 items -->
<div *ngIf="show">
<div *ngFor="let subitem of item.subitems" [@fadeOut]> <!-- 20 each, 200 total -->
{{subitem.name}}
</div>
</div>
</div>
```
Whenever I set `show` to false, it takes too long to rerender and application is frozen for the period of time. If I remove the first `fadeOut` animation, everything works faster and it works perfectly only if I remove even the last animation.
I looked into it for a while and discovered that the problem lies in calling functinon `WebAnimationsDriver#computeStyle` as shown in the screenshot below:

Call stack of the function:
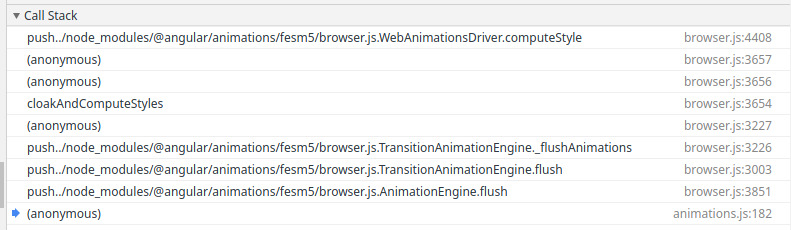
The bug prevents me from using animations for lists and I believe that it is quite a big problem.
## ๐ฌ Minimal Reproduction
https://stackblitz.com/edit/angular-issue-repro2-kpusjq?file=src%2Fapp%2Fapp.component.ts
## ๐ Your Environment
**Angular Version:**
<pre><code>
Angular CLI: 7.1.3
Node: 11.5.0
OS: linux x64
Angular: 7.1.3
... animations, cli, common, compiler, compiler-cli, core, forms
... http, language-service, platform-browser
... platform-browser-dynamic, router
Package Version
-----------------------------------------------------------
@angular-devkit/architect 0.11.3
@angular-devkit/build-angular 0.11.4
@angular-devkit/build-optimizer 0.11.4
@angular-devkit/build-webpack 0.11.4
@angular-devkit/core 7.1.3
@angular-devkit/schematics 7.1.3
@angular/cdk 7.2.0
@ngtools/webpack 7.1.4
@schematics/angular 7.1.3
@schematics/update 0.11.3
rxjs 6.3.3
typescript 3.1.6
webpack 4.23.1
</code></pre> | type: bug/fix,area: animations,freq1: low,P3 | low | Critical |
395,573,364 | TypeScript | Allow paths to be masked and captured by regex | <!-- ๐จ STOP ๐จ ๐ฆ๐ง๐ข๐ฃ ๐จ ๐บ๐ป๐ถ๐ท ๐จ
Half of all issues filed here are duplicates, answered in the FAQ, or not appropriate for the bug tracker. Please read the FAQ first, especially the "Common Feature Requests" section.
-->
## Suggestion
Sometimes if we use a monorepo we will construct our monopackages like this:
```
packages
- a
- src/
- index.ts
- tsconfig.json
- package.json
- b
- src/
- index.ts
- tsconfig.json
- package.json
- c
- src/
- index.ts
- hello/
- world.ts
- tsconfig.json
- package.json
```
So when we need to eject it as a git repo it is trivially done. However, I noticed that TypeScript path resolution is pretty limited to just simple replacement, how it is not working will be talked about later below. And I believe my examples are easier than my explanations :(
## Scenario
Using babel-plugin-module-resolver, it was easy as pie:
```js
const moduleResolver = [
'babel-plugin-module-resolver', {
'root': ['.'],
'alias': {
'^@app\/([^\/]+)\/?(.+)?': './packages/\\1/src/\\2'
}
}
]
```
But with the `paths` option of typescript, which only does simple substitution and is very useless that it could only accommodate one pattern star, is obviously much weaker in expressing power and cannot resolve context dependent subdirectories/submodules.
## Examples
Consider this tsconfig
```json
// Trying to use a common pattern to match "@app/{a,b,c}":
"paths": {
"@app/*": ["packages/*/src"]
}
// Fine, what about "@app/c/hello/world"? It is actually substituted as packages/c/hello/world/src!
// Doing a brute-force construction:
"paths": {
"@app/a*": ["packages/a/src", "packages/a/src*"],
"@app/b*": ["packages/b/src", "packages/b/src*"],
"@app/c*": ["packages/c/src", "packages/c/src*"],
}
// It works but it wasn't elegant...consider if you have hundreds of monopackages and this would be hell
// Only if we could using a regex like pattern in babel-plugin-module-resolver:
"paths": {
"^@app\/([^\/]+)\/?(.+)?": ["./packages/\\1/src/\\2"]
}
// Ah, much easier
```
## Checklist
My suggestion meets these guidelines:
* [ ] This wouldn't be a breaking change in existing TypeScript/JavaScript code
* [x] This wouldn't change the runtime behavior of existing JavaScript code
* [x] This could be implemented without emitting different JS based on the types of the expressions
* [x] This isn't a runtime feature (e.g. library functionality, non-ECMAScript syntax with JavaScript output, etc.)
* [x] This feature would agree with the rest of [TypeScript's Design Goals](https://github.com/Microsoft/TypeScript/wiki/TypeScript-Design-Goals).
# Similar issues
https://github.com/Microsoft/TypeScript/issues/27298
https://github.com/Microsoft/TypeScript/issues/26787
| Suggestion,Awaiting More Feedback | medium | Critical |
395,577,264 | godot | Area2D reports TileMap in the signals, when collide with the tiles | **Godot version:**
Godot 3.1-a4
**OS/device including version:**
Linux Mint 19.1
CPU: Intel Pentium G3250 (2) @ 3.200GHz
GPU: NVIDIA GeForce GTX 750
**Issue description:**
Area2D, when overlapping with the TileMap, sent a signals (body_centered, body_exited, body_shape_entered, body_shape_exited). But as the 'body' argument sends a TileMap. Maybe instead it should sent StaticBody2D / KinematicBody2D depending on the settings of the TileMap?
**Steps to reproduce:**
Collide Area2D and Tile with CollisionShape. Area2D signals will contain TileMap.
**Minimal reproduction project:**
[CollideArea2DAndTileMap.zip](https://github.com/godotengine/godot/files/2723855/CollideArea2DAndTileMap.zip)
| bug,confirmed,topic:physics | low | Major |
395,629,044 | go | x/tools/go/packages: misleading error messages | go list is sensitive to GOPATH, but the error message do not tell the user what to do.
In particular
go list -deps=true -- ~/gostuff/foo
fails with "cannot import absolute path" if GOPATH doesn't include ~/gostuff, but works if it does.
| NeedsInvestigation,Tools | low | Critical |
395,640,344 | go | syscall: sort environment passed to CreateProcess / CreateProcessAsUser | <!-- Please answer these questions before submitting your issue. Thanks! -->
### What version of Go are you using (`go version`)?
<pre>
go version go1.11.2 darwin/amd64
</pre>
### Does this issue reproduce with the latest release?
Yes.
### What operating system and processor architecture are you using (`go env`)?
<details><summary><code>go env</code>Output</summary><br><pre>
$ go env
GOARCH="amd64"
GOBIN=""
GOCACHE="/Users/pmoore/Library/Caches/go-build"
GOEXE=""
GOFLAGS=""
GOHOSTARCH="amd64"
GOHOSTOS="darwin"
GOOS="darwin"
GOPATH="/Users/pmoore/go"
GOPROXY=""
GORACE=""
GOROOT="/usr/local/Cellar/go/1.11.2/libexec"
GOTMPDIR=""
GOTOOLDIR="/usr/local/Cellar/go/1.11.2/libexec/pkg/tool/darwin_amd64"
GCCGO="gccgo"
CC="clang"
CXX="clang++"
CGO_ENABLED="1"
GOMOD=""
CGO_CFLAGS="-g -O2"
CGO_CPPFLAGS=""
CGO_CXXFLAGS="-g -O2"
CGO_FFLAGS="-g -O2"
CGO_LDFLAGS="-g -O2"
PKG_CONFIG="pkg-config"
GOGCCFLAGS="-fPIC -m64 -pthread -fno-caret-diagnostics -Qunused-arguments -fmessage-length=0 -fdebug-prefix-map=/var/folders/v9/mll6p_rj5h94dt_m5m8j0f9c0000gn/T/go-build797508603=/tmp/go-build -gno-record-gcc-switches -fno-common"
</pre></details>
### What did you do?
I looked at https://github.com/golang/go/blob/go1.11.4/src/syscall/exec_windows.go#L97-L122
### What did you expect to see?
I expected to see the env vars [sorted alphabetically by name, with case-insensitive sort, Unicode order, without regard to locale](https://docs.microsoft.com/en-us/windows/desktop/ProcThread/changing-environment-variables).
### What did you see instead?
The code does not sort the environment variable entries. The [MSDN docs](https://docs.microsoft.com/en-us/windows/desktop/ProcThread/changing-environment-variables) state:
> All strings in the environment block must be sorted alphabetically by name. The sort is case-insensitive, Unicode order, without regard to locale. Because the equal sign is a separator, it must not be used in the name of an environment variable.
Note, this hasn't caused me any problems - but it seems like this could cause problems with any Windows kernel functions that expect the env to be sorted. | NeedsInvestigation,early-in-cycle | low | Critical |
395,667,590 | rust | Can you make "somestr".trim_matches(char::is_ascii_punctuation) compile too ? it compiles with char::is_numeric | this works:
```rust
assert_eq!("123foo1bar123".trim_matches(char::is_numeric), "foo1bar"); //ok
```
but this doesn't:
```rust
assert_eq!(
".,\"foo1bar\".,';".trim_matches(char::is_ascii_punctuation),
"foo1bar"
); //XXX fail
// expected signature of `fn(char) -> _`
// found signature of `for<'r> fn(&'r char) -> _`
// = note: required because of the requirements on the impl of `std::str::pattern::Pattern<'_>` for `for<'r> fn(&'r char) -> bool {std::char::methods::<impl char>::is_ascii_punctuation}`
```
sig for [is_numeric](https://github.com/rust-lang/rust/blob/79d8a0fcefa5134db2a94739b1d18daa01fc6e9f/src/libcore/char/methods.rs#L737) is:
```rust
pub fn is_numeric(self) -> bool
```
And for [is_ascii_punctuation](https://github.com/rust-lang/rust/blob/79d8a0fcefa5134db2a94739b1d18daa01fc6e9f/src/libcore/char/methods.rs#L1276) is:
```rust
pub fn is_ascii_punctuation(&self) -> bool
```
So in order to make that work, I've had to do this:
```rust
pub trait Man {
fn manual_is_ascii_punctuation(self) -> bool;
}
impl Man for char {
#[inline]
fn manual_is_ascii_punctuation(self) -> bool {
self.is_ascii() && (self as u8).is_ascii_punctuation()
}
}
fn main() {
assert_eq!(
".,\"foo1bar\".,';".trim_matches(char::manual_is_ascii_punctuation),
"foo1bar"
); //works because the func sig matches
}
```
Full code ([playground](https://play.rust-lang.org/?version=nightly&mode=debug&edition=2018&gist=755046954fcc672185f40cc2c1c0ff8a)):
```rust
#![allow(unused)]
pub trait Man {
fn manual_is_ascii_punctuation(self) -> bool;
}
impl Man for char {
#[inline]
fn manual_is_ascii_punctuation(self) -> bool {
self.is_ascii() && (self as u8).is_ascii_punctuation()
}
}
fn main() {
assert_eq!("11foo1bar11".trim_matches('1'), "foo1bar");
assert_eq!("123foo1bar123".trim_matches(char::is_numeric), "foo1bar"); //ok
assert_eq!(
".,\"foo1bar\".,';".trim_matches(char::manual_is_ascii_punctuation),
"foo1bar"
); //works because the func sig matches
assert_eq!(
".,\"foo1bar\".,';".trim_matches(char::is_ascii_punctuation),
"foo1bar"
); //XXX fail
// expected signature of `fn(char) -> _`
// found signature of `for<'r> fn(&'r char) -> _`
// = note: required because of the requirements on the impl of `std::str::pattern::Pattern<'_>` for `for<'r> fn(&'r char) -> bool {std::char::methods::<impl char>::is_ascii_punctuation}`
assert_eq!("\"123foo1bar\"".trim_matches(|x| x == '"'), "123foo1bar"); //ok
let x: &[_] = &['1', '2'];
assert_eq!("12foo1bar12".trim_matches(x), "foo1bar");
}
```
```rust
Compiling playground v0.0.1 (/playground)
error[E0631]: type mismatch in function arguments
--> src/main.rs:22:29
|
22 | ".,\"foo1bar\".,';".trim_matches(char::is_ascii_punctuation),
| ^^^^^^^^^^^^
| |
| expected signature of `fn(char) -> _`
| found signature of `for<'r> fn(&'r char) -> _`
|
= note: required because of the requirements on the impl of `std::str::pattern::Pattern<'_>` for `for<'r> fn(&'r char) -> bool {std::char::methods::<impl char>::is_ascii_punctuation}`
error: aborting due to previous error
For more information about this error, try `rustc --explain E0631`.
error: Could not compile `playground`.
To learn more, run the command again with --verbose.
```
| C-enhancement,T-libs-api | low | Critical |
395,691,624 | go | x/text/encoding: Go's reuse of character sets causes incorrect decoding of invalid input | <!-- Please answer these questions before submitting your issue. Thanks! -->
### What version of Go are you using (`go version`)?
<pre>
$ go version
go version go1.11 linux/amd64
</pre>
### Does this issue reproduce with the latest release?
Yes
### What operating system and processor architecture are you using (`go env`)?
amd64, linux
### What did you do?
1. Call charset.Lookup("us-ascii") to get an encoding
https://godoc.org/golang.org/x/net/html/charset#Lookup
e, _ := charset.Lookup("us-ascii")
2. Call encoding.NewDecoder().String(string([]byte{0x80})) and get the result
res, _ := e.NewDecoder().String(string([]byte{0x80}))
3. Print the result
### What did you expect to see?
I expect to see a ๏ฟฝ character, since the value is outside of the range of US-ASCII (most significant bit is 1)
### What did you see instead?
I will see the character โฌ from the Windows 1252 encoding instead. This is caused because go is re-using Windows 1252 for US-ASCII. Similar issues arise for out of bounds characters in other charactersets, for example tis-620 maps to windows874. Now if I want to correctly parse the text I need to read through the decoded runes and test if any of them are out of bounds. If I want to use windows874 for just tis-620 characters, I would have to do a similar manual exclusion of out of bounds characters. I do not know of a way to create my own characterset so that these problems can be avoided.
| NeedsInvestigation | low | Minor |
395,743,028 | go | cmd/compile: simplify converting a Go function to buildable Go assembly | I'm slowly learning x86 assembly and how Go uses it, so it's very useful to see how existing functions in Go are translated to it.
The next logical step is to be able to make small changes to that assembly, to see if my understanding of it is correct, and to play with trying to make the code better manually. However, as I've found out, there's no easy way to move a function from a `.go` file to a `.s` file.
After reading some blog posts online and some trial and error, I've found out that most functions can be translated by hand. For example, take a single file with this very simple Go function:
```
package p
func Add(x, y int) int {
return x + y
}
```
With `go tool compile -S f.go`, we can scroll through the output to find its assembly:
```
TEXT "".Add(SB), NOSPLIT|ABIInternal, $0-24
FUNCDATA $0, gclocalsยท33cdeccccebe80329f1fdbee7f5874cb(SB)
FUNCDATA $1, gclocalsยท33cdeccccebe80329f1fdbee7f5874cb(SB)
FUNCDATA $3, gclocalsยท33cdeccccebe80329f1fdbee7f5874cb(SB)
PCDATA $2, $0
PCDATA $0, $0
MOVQ "".y+16(SP), AX
MOVQ "".x+8(SP), CX
ADDQ CX, AX
MOVQ AX, "".~r2+24(SP)
RET
```
However, we can't just add the assembly to the source code and make the Go func a stub, as that just fails:
```
$ go build
# foo.bar
./f.s:1: string constant must be an immediate
./f.s:7: string constant must be an immediate
./f.s:8: string constant must be an immediate
./f.s:10: string constant must be an immediate
asm: assembly of ./f.s failed
```
We can apply multiple changes to get it to work:
* remove `FUNCDATA` and `PCDATA` lines
* replace `"".Func` with `ยทFunc`
* remove all addressing modes (?) like `NOSPLIT|ABIInternal`, to get rid of errors like `illegal or missing addressing mode for symbol NOSPLIT`
* replace `"".var` with `var`
* replace `"".~r2` with `r2` (I still don't understand that tilde)
End result, which works just as fine as the Go code:
```
TEXT ยทAdd(SB),$0-24
MOVQ y+16(SP), AX
MOVQ x+8(SP), CX
ADDQ CX, AX
MOVQ AX, r2+24(SP)
RET
```
In this case, we're done. But if the function had jumps like `JMP 90`, we'd have to add a label like `L90` after finding the right line, and then modifying the jump to be `JMP L90`. `go vet` also tends to complain about the result of the process above, even when the assembly works - for example, in our case:
```
$ go vet
# foo.bar
./f.s:6:1: [amd64] Add: RET without writing to 8-byte ret+16(FP)
```
I've succsesfully done this with multiple funcs on the small side, but it's tedious. Worse even, I still haven't been able to correctly translate a function calling another function to assembly - I keep getting panics like `runtime: unexpected return pc for ...`, which are very confusing.
I think the compiler should support translating Go functions to assembly which can just be dumped onto a `.s` file after replacing their Go implementation with a stub. It doesn't matter if it couldn't directly work on some weird edge cases; if at least it could give a starting point after doing grunt work as mentioned above, that would already be a huge improvement.
Ideas that come to mind to improve `go tool compile -S` (thanks for @josharian for his input):
* Allow filtering for functions, e.g. via a regexp. Similar to `GOSSAFUNC`.
* Add a mode where the output can theoretically/probably be built inside a `.s` file as-is.
For example, borrowing from flags like `compile -d`, one might imagine `go tool compile -S=func=Add,clean` to do what I had done manually above.
If this makes sense, I'm happy to do some of the work during the upcoming cycle. I'll just need a bit of help from the compiler folks, as my assembly (and especially Go assembly syntax) knowledge is limited.
/cc @randall77 @griesemer @josharian @mdempsky @martisch, as per https://dev.golang.org/owners/. | NeedsDecision,FeatureRequest,compiler/runtime | medium | Critical |
395,759,070 | pytorch | Nn.dataparallel with multiple output, weird gradient result None | ## ๐ Bug
Under PyTorch 1.0, nn.DataParallel() wrapper for models with multiple outputs does not calculate gradients properly.
## To Reproduce
On servers with >=2 GPUs, under PyTorch 1.0.0
Steps to reproduce the behavior:
1. Use the code in below:
```
import torch.nn as nn
import torch
import torch.nn.functional as F
DEVICE = torch.device('cuda:0')
class NN4(nn.Module):
def __init__(self):
super(NN4, self).__init__()
self.fc1 = nn.Linear(8, 4)
self.fc21 = nn.Linear(4, 1)
def forward(self, x):
x = F.selu(self.fc1(x))
x1 = torch.sigmoid(self.fc21(x))
# return x, x # not None
return x, x1 # None
def test_NN4():
images = torch.randn(4, 8).to(DEVICE)
fimages = torch.randn(4, 8).to(DEVICE)
D = NN4().to(DEVICE)
D = nn.DataParallel(D)
D.zero_grad()
d_loss = D(images)[0].mean() - D(fimages)[0].mean()
print('d_loss: -->', d_loss)
d_loss.backward()
print('-------->>>')
aaa = list(D.named_parameters())
print(aaa[0][0])
print(aaa[0][1].grad)
D2 = NN4().to(DEVICE)
D2.zero_grad()
d2_loss = D2(images)[0].mean() - D2(fimages)[0].mean()
print('d2_loss: -->', d2_loss)
d2_loss.backward()
print('-------->>>')
aaa2 = list(D2.named_parameters())
print(aaa2[0][0])
print(aaa2[0][1].grad)
```
Then run the code with "CUDA_VISIBLE_DEVICES=0,1 python dp_test.py" in console. Under PyTorch 1.0.0, I get:
```
d_loss: --> tensor(0.1488, device='cuda:0', grad_fn=<SubBackward0>)
-------->>>
module.fc1.weight
None
d2_loss: --> tensor(0.0149, device='cuda:0', grad_fn=<SubBackward0>)
-------->>>
fc1.weight
tensor([[ 0.0284, -0.1972, 0.1553, -0.3356, 0.2737, -0.2083, 0.1420, -0.3533],
[ 0.0473, -0.1277, 0.0903, -0.3214, 0.2385, -0.1815, 0.0369, -0.1991],
[ 0.0231, -0.0949, 0.1218, -0.3591, 0.1832, -0.2311, 0.0685, -0.1934],
[ 0.0858, -0.1129, 0.1216, -0.3774, 0.3795, -0.1308, -0.0006, -0.1790]],
device='cuda:0')
```
However, under PyTorch 0.4.0, I get:
```
d_loss: --> tensor(0.1650, device='cuda:0')
-------->>>
module.fc1.weight
tensor([[-0.2463, 0.0740, -0.2929, -0.2576, -0.0346, 0.1679, 0.1501,
-0.2375],
[-0.2666, 0.1135, -0.3788, -0.2865, -0.0519, -0.0217, 0.0564,
-0.2942],
[-0.2802, 0.1207, -0.3556, -0.2959, -0.0245, -0.0106, 0.0902,
-0.2851],
[-0.3193, 0.0788, -0.4258, -0.2705, -0.1212, 0.0063, 0.0322,
-0.2649]], device='cuda:0')
d2_loss: --> tensor(1.00000e-02 *
8.7814, device='cuda:0')
-------->>>
fc1.weight
tensor([[-0.3051, 0.1011, -0.3452, -0.2829, -0.0318, -0.0299, 0.0642,
-0.2442],
[-0.2536, 0.1279, -0.3869, -0.3891, -0.0362, 0.0412, 0.1000,
-0.3384],
[-0.3321, 0.0059, -0.4514, -0.2517, -0.1013, 0.0374, 0.0124,
-0.1985],
[-0.3147, 0.0331, -0.3343, -0.2498, -0.0903, -0.0668, 0.0555,
-0.2360]], device='cuda:0')
```
## Expected behavior
`aaa[0][1].grad` should not be none under PyTorch 1.0.0
## Environment
PyTorch version: 1.0.0
Is debug build: No
CUDA used to build PyTorch: 9.0.176
OS: Ubuntu 16.04.5 LTS
GCC version: (Ubuntu 5.4.0-6ubuntu1~16.04.10) 5.4.0 20160609
CMake version: version 3.5.1
Python version: 3.6
Is CUDA available: Yes
CUDA runtime version: 9.0.176
GPU models and configuration:
GPU 0: Tesla V100-SXM2-16GB
GPU 1: Tesla V100-SXM2-16GB
GPU 2: Tesla V100-SXM2-16GB
GPU 3: Tesla V100-SXM2-16GB
Nvidia driver version: 396.44
cuDNN version: Probably one of the following:
/usr/local/cuda-8.0/lib64/libcudnn.so.6.0.21
/usr/local/cuda-8.0/lib64/libcudnn_static.a
/usr/local/cuda-9.0/lib64/libcudnn.so.7.3.1
/usr/local/cuda-9.0/lib64/libcudnn_static.a
/usr/local/cuda-9.1/lib64/libcudnn.so.7.0.5
/usr/local/cuda-9.1/lib64/libcudnn_static.a
/usr/local/cuda-9.2/lib64/libcudnn.so.7.3.1
/usr/local/cuda-9.2/lib64/libcudnn_static.a
## Additional context
<!-- Add any other context about the problem here. -->
| oncall: distributed,triaged | low | Critical |
395,782,593 | godot | External text editor does not open unless Godot quits | <!-- Please search existing issues for potential duplicates before filing yours:
https://github.com/godotengine/godot/issues?q=is%3Aissue
-->
**Godot version:**
<!-- Specify commit hash if non-official. -->
3.2 branch at commit 68013d23932688e57b489600f4517dd280edc464
**OS/device including version:**
<!-- Specify GPU model and drivers if graphics-related. -->
Arch Linux x86_64 - gcc (GCC) 8.2.1 20181127
**Issue description:**
<!-- What happened, and what was expected. -->
After trying to run the Finite State Machine example in the demos, which currently has faulty GDScript code, I expected my external editor to run but this was not the case. I placed some debugging code in `OS_Unix::execute` and turns out that the `execvp` attempt is actually another command:
```
Attempting to execute: /opt/godot/bin/godot.x11.opt.tools.64 --path /home/wilson/gitrepos/godot-demo-projects/2d/finite_state_machine --remote-debug 127.0.0.1:6007 --allow_focus_steal_pid 1863 --position 320,180 (null)
```
For some reason, whether the project was not stopped (i.e. if F8 is not pressed) or not, and Godot quits, the next time Godot opens, it attempts to run my external editor:
```
Attempting to execute: xterm -bg black -fg white -sl 100000 -e vim +"call cursor(0, 0) /home/wilson/gitrepos/godot-demo-projects/2d/finite_state_machine/player/weapon/sword.gd (null)
```
For some reason I also had to escape that `+` symbol with a backslash `\` in the argument list within the editor.
For what is worth, here's the code I placed right before the `execvp` call in `OS_Unix::execute`:
```cpp
fprintf(stderr, "Attempting to execute:");
for (int i = 0; i < args.size(); i++)
fprintf(stderr, " %s", args[i]);
fprintf(stderr, "\n");
```
**Steps to reproduce:**
- Change external editor after opening project using `godot -e` from within the project path
- Attempt to run Finite State Machine from the [official demos](https://github.com/godotengine/godot-demo-projects/tree/master/2d/finite_state_machine) (press F5), if the scene runs fine, place some faulty GDScript code.
- When the faulty GDScript code is detected, the external editor should have opened at the offending line but it doesn't.
- Close Godot
- Reopen the project and attempt to run Finite State Machine demo again after opening project using `godot -e` from within the project path, the external editor still doesn't open after detecting the faulty GDScript code.
**Minimal reproduction project:**
See the Finite State Machine example in the `godot-demo-repository` repository at commit eb27a35a2d5212abfc516aa3c8fcb76c907155f0
<!-- Recommended as it greatly speeds up debugging. Drag and drop a zip archive to upload it. -->
| bug,topic:core,confirmed | low | Critical |
395,782,893 | vscode | Scroll bar visibility should be configurable | ### VSCode Version
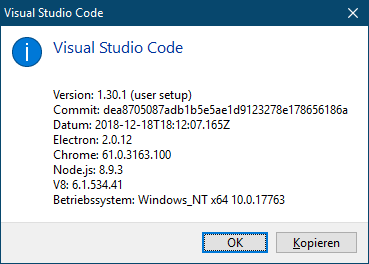
### Steps to Reproduce
1. Open Visual Studio code and open a large text file
### Current Behaviour
In Visual Studio Code, editors' scroll bars auto hide, thereby thwarting targeting the scroll bar handle when moving the mouse.
### Expected Behaviour
Visual Studio Code should follow the Windows system setting `Settings > Ease of Access > Display > Automatically hide scrollbar in Windows`
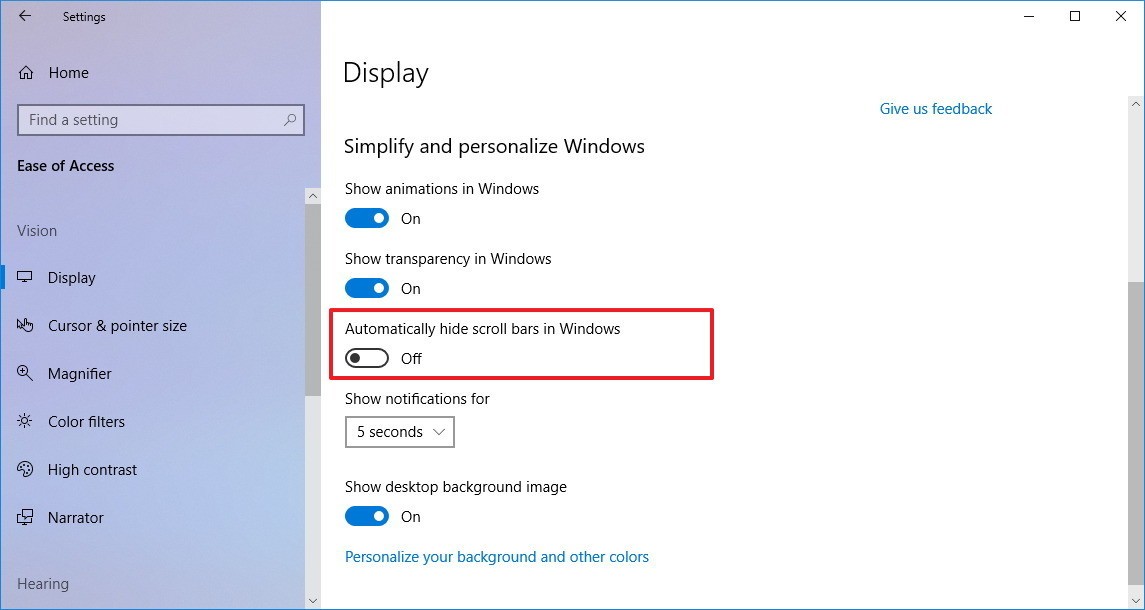
### Does this issue occur when all extensions are disabled?
- [x] Yes
- [ ] No
| feature-request,workbench-os-integration | medium | Critical |
395,866,585 | vscode | Intellisense's 'Suggest selection' should keep working when you have typed something | VSCode has a feature that remembers recent completions the user has selected and then automatically choosen those completions for them the next time.
The problem is that it behaves quite oddly, and here is an example that illustrates why:
- Create a new Typescript file.
- Type `console.` vscode will suggest all of the available methods of `console`.
- Choose `exception`.
- Type `console.` again. Vscode will immediately suggest `exception`, as expected.
And now here is the weird part: Type `e`, and instead of still suggesting `exception`, it will suggest `error`!
The problem here is that once you type anything after the suggestion came, vscode will completely disregard your completion history and will just give you the best match lexicographically. Even if in the example you have completed `exception` by typing an `e` and then having it complete it, the same thing will occur.
My suggestion is that when you have already typed something, the 'suggest selection' will just be filtered by what you have typed, instead of stop working. So in the example, after you have typed `e`, it will still suggest `exception`.
_"Switch the Suggest Selection settings to "recentlyUsedByPrefix" and it will work!"_
Yes it does make it sometimes work but overall it makes the situation much worse. For example if you type just `console.` it won't suggest exception no matter what you have done before.
| feature-request,suggest,under-discussion | low | Critical |
395,933,053 | go | x/tools/go/packages: unhelpful when it returns nil, nil | at tip, packages.Load can return an empty list without any errors. While this might be the correct result sometimes (as when a pattern doesn't match) it gives the user nothing to work with. It might be more helpful to report some sort of error from the underlying build tool.
| NeedsInvestigation,Tools | low | Critical |
395,949,709 | angular | Dot segments from path not removed | # ๐ bug report
### Affected Package
@angular/router
### Is this a regression?
No
### Description
By RFC3986 (https://tools.ietf.org/html/rfc3986#page-33) dot segments should be removed from path.
When accessing `localhost:4200/segment/./segment` by browser navigator, browser strips the dot segments and Angular router recieves `localhost:4200/segment/segment`. Everything works as expected.
When programmatically routing to `'./segment/./segment'`, Angular throws an error (404).
Browser navigator still shows URI with dot segments *stripped* (`localhost:4200/segment/segment`).
## ๐ฌ Minimal Reproduction
https://stackblitz.com/edit/angular-d2kk5q?file=src%2Findex.html
## ๐ฅ Exception or Error
Cannot match any routes. URL Segment: 'segment/./segment'
## ๐ Your Environment
**Angular Version:**
7.0.1
## Extra
I'm not sure if I would call this a bug, but it's definitely not consistent with browser routes.
| type: bug/fix,freq1: low,area: router,state: confirmed,router: URL parsing/generation,P5 | low | Critical |
395,965,550 | go | x/tools/go/packages/packagestest: cleanup fails silently and leaves test junk | The `Exported.Cleanup` function from `x/tools/go/packages/packagestest` can fail silently and leave temporary files around - currently running `go test` in `x/tools/import` results in around 75MB of test junk being left behind (at least under OpenBSD and I would suspect most other BSD/Unix like platforms), the majority being from `TestSimpleCases`.
The cause of the issue is that one of the sub-directories is created as 0555, which means that the subsequent `os.RemoveAll` call fails due to insufficient permissions:
```
$ ls -l /tmp/TestSimpleCases_import_grouping_not_path_dependent_no_groups_Modules592366644/modcache/pkg/mod/
total 20
drwxr-xr-x 6 joel joel 512 Jan 1 23:53 github.com/
drwxr-xr-x 3 joel joel 512 Jan 1 23:53 golang.org/
dr-xr-xr-x 3 joel joel 512 Jan 1 23:53 [email protected]/
dr-xr-xr-x 6 joel joel 512 Jan 1 23:53 [email protected]/
dr-xr-xr-x 3 joel joel 512 Jan 1 23:53 [email protected]/
```
There are possibly three separate issues here:
1. Either these directories need to be created with permissions 0755, or the `Exported.Cleanup` function needs to `chmod u+w` before trying to `os.RemoveAll`.
2. The `Exported.Cleanup` function should not really fail silently, since these kinds of issues go undetected until a disk fills up.
3. There may be an issue with the code that is creating these directories (which should be using 0755 instead of 0555), if it is outside the test harness. | NeedsInvestigation,Tools | low | Minor |
395,973,607 | go | cmd/go: clean GOCACHE based on disk usage | The GOCACHE appears to lack a disk size limit - this is a problem in a space constrained environment and/or when running go on a disk that is nearing capacity. For example, on the openbsd/arm builder (which runs on a USB stick), the `~/.cache/go-build` directory runs past several GB in a very short time, which then leads to various failures (`git clone` or go builds). The only option that I currently appear to have is to run `go cache -clean` regularly, in order to keep the cache at a respectable size. It seems that having a configurable upper bound would be preferable and/or free disk space based checks that prevent writes (e.g. evict then write) to the cache from failing when the disk is full due, partly due to a large GOCACHE:
```
[gopher@cubox ~ 102]$ du -csh ~/.cache/go-build
2.2G /home/gopher/.cache/go-build
2.2G total
[gopher@cubox ~ 103]$ df -h /home
Filesystem Size Used Avail Capacity Mounted on
/dev/sd1m 9.0G 8.5G -6.0K 100% /home
[gopher@cubox ~ 104]$ go build -o /tmp/main /tmp/main.go
/home: write failed, file system is full
/home: write failed, file system is full
/home: write failed, file system is full
/home: write failed, file system is full
/home: write failed, file system is full
/home: write failed, file system is full
/home: write failed, file system is full
/home: write failed, file system is full
/home: write failed, file system is full
[gopher@cubox ~ 105]$ du -csh ~/.cache/go-build
2.2G /home/gopher/.cache/go-build
2.2G total
[gopher@cubox ~ 106]$ df -h /home
Filesystem Size Used Avail Capacity Mounted on
/dev/sd1m 9.0G 8.5G -6.0K 100% /home
``` | NeedsDecision,FeatureRequest,GoCommand | medium | Critical |
395,999,244 | TypeScript | "Pick" or "Exclude" constructor from class type | <!-- ๐จ STOP ๐จ ๐ฆ๐ง๐ข๐ฃ ๐จ ๐บ๐ป๐ถ๐ท ๐จ
Half of all issues filed here are duplicates, answered in the FAQ, or not appropriate for the bug tracker. Please read the FAQ first, especially the "Common Feature Requests" section.
-->
## Search Terms
`intersect`, `type`, `intersect constructor`, `pick new`, `pick constructor`
<!-- List of keywords you searched for before creating this issue. Write them down here so that others can find this suggestion more easily -->
## Suggestion
```ts
class C1 {
static S1 = 'foo';
bar = 'bar';
constructor(p1, p2, p3) {}
}
type T1 = Pick<typeof C1, new>;
// could simply be also:
type Only<T, K extends keyof T> = Pick<T, { [P in keyof T]: P extends K ? P : never }[keyof T]>;
type T2 = Only<typeof C1, 'constructor'>;
const clazz: T1 = null; // `null` could be anything
new clazz(); // should throw error because of missing params
new clazz(null, null, null); // should work
new clazz(null, null, null).bar; // should throw error because `bar` doesn't exist
clazz.S1; // should throw error because `S1` doesn't exist
```
## Use Cases
It could be useful in class type intersection and/or for multiple inheritance (like PHP Trait, or Java default methods in interface).
And make mixins more "clean" imho.
One of my goal is to make a "type mixin" without a "value mixin".
## Examples
For example:
```ts
class C1 {
public bar: string;
constructor(p1: string) {}
public foo() {}
}
class C2 {
public constructor() {}
public baz() {}
}
type T1 = typeof C1 & typeof C2;
const a: T1 = null;
a.prototype.foo(); // ok
a.prototype.bar; // ok
a.prototype.baz(); // ok
new a(); // ok (return C2)
new a(''); // ok (return C1)
new a().foo() // error
new a('').baz(); // error
type T2 = typeof C1 & Exclude<typeof C2, new>
const b: T2 = null;
b.prototype.foo(); // ok
b.prototype.bar; // ok
b.prototype.baz(); // ok
new a(); // error <<<
new a(''); // ok (return C1 with C2 prototype)
new a('').foo() // ok (from C1)
new a('').baz(); // ok (from C2) <<<
```
## Checklist
My suggestion meets these guidelines:
* [x] This wouldn't be a breaking change in existing TypeScript/JavaScript code
* [x] This wouldn't change the runtime behavior of existing JavaScript code
* [x] This could be implemented without emitting different JS based on the types of the expressions
* [ ] This isn't a runtime feature (e.g. library functionality, non-ECMAScript syntax with JavaScript output, etc.)
* [x] This feature would agree with the rest of [TypeScript's Design Goals](https://github.com/Microsoft/TypeScript/wiki/TypeScript-Design-Goals).
| Suggestion,Needs Proposal | low | Critical |
396,007,087 | pytorch | Beta Distribution values wrong for a=b---> 0 | ## ๐ Bug
#### When concentration parameters a, b are equal and go to 0, sample values are wrong.
## To Reproduce
Steps to reproduce the behavior:
copy paste the following in a notebook:
```
import torch
from scipy.stats import beta as beta_sp
from torch.distributions import Beta as beta_t
from matplotlib import pyplot as plt
device = torch.device('cuda:0' if torch.cuda.is_available() else 'cpu')
dtype = torch.float32
a = 1e-2
b = 1e-2
size = [10000]
x1 = beta_sp.rvs(a, b, size=size)
x2 = beta_t(torch.tensor(a, device=device, dtype=dtype),
torch.tensor(b, device=device, dtype=dtype)).sample(torch.Size(size))
def plot_compare(x1, x2):
plt.hist([x1, x2.cpu().numpy()], label=['scipy', 'pytorch'], density=True, alpha=0.75);
plt.legend(loc='best')
plt.title('Beta distribution for values a=b=%.3f' % a)
plt.show()
# wrong as a=b --> 0, starting at 1e-2
plot_compare(x1, x2)
```
(1) for `device = cpu`, there is an error peak around 0.55
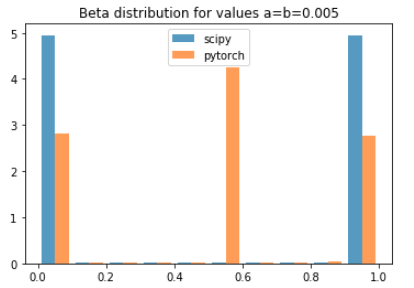
(2) for `dvice = cuda`, there is a bias towards 1.
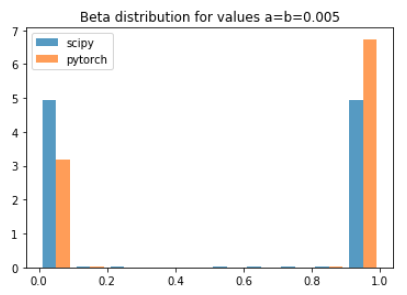
## Expected behavior
see the `scipy` output for expected values.
## Environment
- PyTorch Version (e.g., 1.0): 1.0
- OS (e.g., Linux): mac
- How you installed PyTorch: `conda`
- Python version: 3.6
- CUDA/cuDNN version: 9.0
- GPU models and configuration: TitanX
cc @vincentqb @fritzo @neerajprad @alicanb @vishwakftw | module: distributions,triaged | medium | Critical |
396,017,184 | flutter | Bad error message when Android toolchain isn't installed | If the Android toolchain isn't installed (I had the wrong path set in `ANDROID_HOME`),
`flutter run` and `flutter devices` report
```
No devices detected
```
instead of
```
Can't find adb / Android tools.
``` | platform-android,tool,P2,team-android,triaged-android | low | Critical |
396,039,706 | create-react-app | HTTPS in Development | A workaround has been commented below, see: https://github.com/facebook/create-react-app/issues/6126#issuecomment-570763433
---
Right now there's just the general acceptance that the SSL Certificate will cause some friction.
> Note that the server will use a self-signed certificate, so your web browser will almost definitely display a warning upon accessing the page.
>
> Source: [Create React App Website](https://facebook.github.io/create-react-app/docs/using-https-in-development)
React's website (and the development world really) has been gifted with [Gatsby](https://github.com/gatsbyjs/gatsby), and I think they got it right.
When you run a gatsby website in development mode with the `--https` flag, it will leverage the package [devcert-san](https://github.com/davewasmer/devcert). It's (near) frictionless, handles trusting the CA and gets you a proper lock on the browser bar.
I don't think it's really a high priority but wanted to bring it up.
> Just to follow up, I also found this but haven't worked with it (yet).
>
> https://github.com/FiloSottile/mkcert
---
**EDIT:**
> a PR implementing this feature has been open for a long time now: #5845 | issue: proposal | medium | Critical |
396,046,933 | go | x/build/cmd/gerritbot: auto-reviewers are not applied for some GitHub CLs | When a PR is created by an author that has a Gerrit account, they are added as a reviewer to their own CL. Since the CL has a reviewer, gerritbot then does not apply autoreviewers.
For example: https://go-review.googlesource.com/c/go/+/156297
Maybe the answer is to add them as CC, not as reviewers. I'm not sure why that's the current behavior, but it might be an automatic Gerrit behavior, similarly to how it adds reviewers if it detects them listed manually as Reviewed-By.
/cc @andybons | help wanted,Builders,NeedsFix | low | Minor |
396,047,514 | vscode | [css][html] SVG language support | Currently, we have no SVG support:
- In HTML, SVG tags don't receive auto completion
- In CSS, SVG attributes don't receive auto completion
- Syntax highlighting are problematic for both SVG tags/attributes in HTML/CSS
- Emmet do not get SVG completions
However, with custom tag/property support in HTML/CSS, we should be able to trivially write a SVG package that describes the set of tags/attributes for SVG, and let HTML/CSS language services do the support work.
- https://github.com/Microsoft/vscode/issues/62976
- https://github.com/Microsoft/vscode/issues/64164 | feature-request,css-less-scss,html | medium | Critical |
396,078,368 | go | cmd/compile: many new no-ops as a result of mid-stack inlining | <!-- Please answer these questions before submitting your issue. Thanks! -->
### What version of Go are you using (`go version`)?
latest
### Does this issue reproduce with the latest release?
yes
### What operating system and processor architecture are you using (`go env`)?
ppc64le and ppc64
### What did you do?
I was inspecting code for another reason and I saw a lot of new no-ops in unexpected places. For example in runtime.cgoCheckTypedBlock:
```
return mheap_.arenas[ai.l1()][ai.l2()].spans[(p/pageSize)%pagesPerArena]
0x13d08 3fe0002b ADDIS $0,$43,R31
0x13d0c e8ff3728 MOVD 14120(R31),R7
0x13d10 8be70000 MOVBZ 0(R7),R31
s := spanOfUnchecked(uintptr(src))
0x13d14 7c000378 OR R0,R0,R0
0x13d18 7c000378 OR R0,R0,R0
0x13d1c 7dc87378 OR R14,R14,R8
ai := arenaIndex(p)
0x13d20 7c000378 OR R0,R0,R0
0x13d24 7c000378 OR R0,R0,R0
return mheap_.arenas[ai.l1()][ai.l2()].spans[(p/pageSize)%pagesPerArena]
0x13d28 7c000378 OR R0,R0,R0
0x13d2c 7c000378 OR R0,R0,R0
0x13d30 7c000378 OR R0,R0,R0
0x13d34 7c000378 OR R0,R0,R0
return arenaIdx((p + arenaBaseOffset) / heapArenaBytes)
0x13d38 79c93682 RLDICL R14,$38,$26,R9
```
### What did you expect to see?
I should only see no-ops where they are expected.
### What did you see instead?
Lots of no-ops in unexpected places. In the test for bytes there are almost 9000 extra no-ops with this commit.
>cd src/bytes
>go test -c
objdump -D bytes.test | grep mr | grep r0,r0 | wc -l
Using go version devel +c043fc4 Fri Dec 28 04:17:55 2018 +0000 linux/ppc64le
347
Using go version devel +69c2c56 Fri Dec 28 20:55:36 2018 +0000 linux/ppc64le
9193
I have not found runtime breakage because of this but performance could be affected depending where the unnecessary no-ops occur. | NeedsFix | low | Major |
396,089,045 | go | cmd/compile: reuse boxed primitive in slice literals of interfaces | Using `go1.12`
Consider the following snippet:
```go
func main() {
lit := []interface{}{
0, 0, 0, 0, 0,
}
type ifaceHeader struct{ Type, Data unsafe.Pointer }
for i := range lit {
fmt.Printf("%+v\n", *(*ifaceHeader)(unsafe.Pointer(&lit[i])))
}
}
```
This currently prints:
```
{Type:0x4962e0 Data:0x4c95c0}
{Type:0x4962e0 Data:0x4c95c8}
{Type:0x4962e0 Data:0x4c95d0}
{Type:0x4962e0 Data:0x4c95d8}
{Type:0x4962e0 Data:0x4c95e0}
```
Which is indicative that each boxed integer is getting it's own heap allocated element (since the data pointer is different for every element). It seems that the compiler should be able to reuse boxed elements that are of the same value.
Furthermore, I would expect this work across different types with the same underlying kind. Thus, I would also expect:
```go
type EnumC int32
type EnumB int32
type EnumC int32
lit := []interface{EnumA(0), EnumB(0), EnumC(0)}
```
to all share the same boxed element since all three types are of the `int32` kind.
\cc @randall77 | Performance,NeedsInvestigation | low | Major |
396,093,262 | kubernetes | ReplicaSet controller continuously creating pods failing due to SysctlForbidden | **What happened**:
Creating a deployment with a pod spec with an unsafe sysctl not whitelisted results in the pods being rejected by Kubelet with `SysctlForbidden` and the replicatset controller just keeps creating new pods in a tight loop.
**What you expected to happen**:
The replicaset controller should maybe backoff exponentially if the pod it tries creating is getting rejected by Kubelet
**How to reproduce it (as minimally and precisely as possible)**:
Add:
```yaml
securityContext:
sysctls:
- name: net.core.somaxconn
value: '10000' # This value really does not matter any int works
```
to a pod spec in a deployment, ensure kubelets in the cluster do not have `allowed-unsafe-sysctls` flag configured.
apply the deployment config and you should see over a 100 pods created under a minute.
**Anything else we need to know?**:
**Environment**:
- Kubernetes version (use `kubectl version`):
```
kubectl version
Client Version: version.Info{Major:"1", Minor:"12", GitVersion:"v1.12.3", GitCommit:"435f92c719f279a3a67808c80521ea17d5715c66", GitTreeState:"clean", BuildDate:"2018-11-26T12:57:14Z", GoVersion:"go1.10.4", Compiler:"gc", Platform:"linux/amd64"}
Server Version: version.Info{Major:"1", Minor:"12", GitVersion:"v1.12.3", GitCommit:"435f92c719f279a3a67808c80521ea17d5715c66", GitTreeState:"clean", BuildDate:"2018-11-26T12:46:57Z", GoVersion:"go1.10.4", Compiler:"gc", Platform:"linux/amd64"}
```
- Cloud provider or hardware configuration: AWS
- OS (e.g. from /etc/os-release): Debian Stretch
- Kernel (e.g. `uname -a`): Linux 4.9
<!-- DO NOT EDIT BELOW THIS LINE -->
/kind bug | kind/bug,priority/important-soon,area/kubelet,sig/scheduling,area/reliability,kind/feature,sig/apps,lifecycle/frozen | high | Critical |
396,115,875 | flutter | Publish iOS fidelity comparison | Once https://github.com/flutter/flutter/projects/9#column-3989168 is done, publish the results (and as a forcing function, make sure it's good enough to be published :D) | platform-ios,framework,a: fidelity,f: cupertino,P3,team-design,triaged-design | low | Minor |
396,144,862 | flutter | On windows, using a ramdisk leads to "Cannot resolve symbolic links" | <!-- Thank you for using Flutter!
If you are looking for support, please check out our documentation
or consider asking a question on Stack Overflow:
* https://flutter.io/
* https://docs.flutter.io/
* https://stackoverflow.com/questions/tagged/flutter?sort=frequent
If you have found a bug or if our documentation doesn't have an answer
to what you're looking for, then fill our the template below. Please read
our guide to filing a bug first: https://flutter.io/bug-reports/
-->
## Steps to Reproduce
<!-- Please tell us exactly how to reproduce the problem you are running into. -->
1. create new flutter app
2. add package, for example 'english_words: ^3.1.5'
3. run 'flutter packages get'
## Logs
<!--
Include the full logs of the commands you are running between the lines
with the backticks below. If you are running any "flutter" commands,
please include the output of running them with "--verbose"; for example,
the output of running "flutter --verbose create foo".
-->
```
flutter packages get --verbose
[ +45 ms] executing: [D:\flutter\] git rev-parse --abbrev-ref --symbolic @{u}
[ +112 ms] Exit code 0 from: git rev-parse --abbrev-ref --symbolic @{u}
[ +3 ms] origin/stable
[ ] executing: [D:\flutter\] git rev-parse --abbrev-ref HEAD
[ +64 ms] Exit code 0 from: git rev-parse --abbrev-ref HEAD
[ ] stable
[ ] executing: [D:\flutter\] git ls-remote --get-url origin
[ +57 ms] Exit code 0 from: git ls-remote --get-url origin
[ ] https://github.com/flutter/flutter.git
[ ] executing: [D:\flutter\] git log -n 1 --pretty=format:%H
[ +61 ms] Exit code 0 from: git log -n 1 --pretty=format:%H
[ ] 5391447fae6209bb21a89e6a5a6583cac1af9b4b
[ ] executing: [D:\flutter\] git log -n 1 --pretty=format:%ar
[ +58 ms] Exit code 0 from: git log -n 1 --pretty=format:%ar
[ ] 5 weeks ago
[ +1 ms] executing: [D:\flutter\] git describe --match v*.*.* --first-parent --long --tags
[ +64 ms] Exit code 0 from: git describe --match v*.*.* --first-parent --long --tags
[ ] v1.0.0-0-g5391447fa
[ +240 ms] Running "flutter packages get" in startup_namer...
[ +4 ms] Using D:\flutter\.pub-cache for the pub cache.
[ +3 ms] executing: [D:\startup_namer\] D:\flutter\bin\cache\dart-sdk\bin\pub.bat --verbosity=warning --verbose get --no-precompile
[+4384 ms] Cannot resolve symbolic links, path = 'H:\Temp\pub_8c3f82f8-10c0-11e9-bee5-60a44c618283' (OS Error: ะะตะฒะตัะฝะฐั ััะฝะบัะธั.
[ +12 ms] , errno = 1)
[ +53 ms] Running "flutter packages get" in startup_namer... (completed)
[ +13 ms] "flutter get" took 4ย 561ms.
pub get failed (66)
#0 throwToolExit (package:flutter_tools/src/base/common.dart:26:3)
#1 pub (package:flutter_tools/src/dart/pub.dart:170:5)
<asynchronous suspension>
#2 pubGet (package:flutter_tools/src/dart/pub.dart:104:13)
<asynchronous suspension>
#3 PackagesGetCommand._runPubGet (package:flutter_tools/src/commands/packages.dart:59:11)
<asynchronous suspension>
#4 PackagesGetCommand.runCommand (package:flutter_tools/src/commands/packages.dart:82:11)
<asynchronous suspension>
#5 FlutterCommand.verifyThenRunCommand (package:flutter_tools/src/runner/flutter_command.dart:401:18)
#6 _asyncThenWrapperHelper.<anonymous closure> (dart:async/runtime/libasync_patch.dart:77:64)
#7 _rootRunUnary (dart:async/zone.dart:1132:38)
#8 _CustomZone.runUnary (dart:async/zone.dart:1029:19)
#9 _FutureListener.handleValue (dart:async/future_impl.dart:129:18)
#10 Future._propagateToListeners.handleValueCallback (dart:async/future_impl.dart:642:45)
#11 Future._propagateToListeners (dart:async/future_impl.dart:671:32)
#12 Future._complete (dart:async/future_impl.dart:476:7)
#13 _SyncCompleter.complete (dart:async/future_impl.dart:51:12)
#14 _AsyncAwaitCompleter.complete.<anonymous closure> (dart:async/runtime/libasync_patch.dart:33:20)
#15 _rootRun (dart:async/zone.dart:1124:13)
#16 _CustomZone.run (dart:async/zone.dart:1021:19)
#17 _CustomZone.bindCallback.<anonymous closure> (dart:async/zone.dart:947:23)
#18 _microtaskLoop (dart:async/schedule_microtask.dart:41:21)
#19 _startMicrotaskLoop (dart:async/schedule_microtask.dart:50:5)
#20 _runPendingImmediateCallback (dart:isolate/runtime/libisolate_patch.dart:115:13)
#21 _RawReceivePortImpl._handleMessage (dart:isolate/runtime/libisolate_patch.dart:172:5)
```
<!-- If possible, paste the output of running `flutter doctor -v` here. -->
```
flutter doctor -v
[โ] Flutter (Channel stable, v1.0.0, on Microsoft Windows [Version 10.0.17763.195], locale ru-RU)
โข Flutter version 1.0.0 at D:\flutter
โข Framework revision 5391447fae (5 weeks ago), 2018-11-29 19:41:26 -0800
โข Engine revision 7375a0f414
โข Dart version 2.1.0 (build 2.1.0-dev.9.4 f9ebf21297)
[โ] Android toolchain - develop for Android devices (Android SDK 28.0.3)
โข Android SDK at D:\AndroidSDK
โข Android NDK location not configured (optional; useful for native profiling support)
โข Platform android-28, build-tools 28.0.3
โข ANDROID_HOME = D:\AndroidSDK
โข Java binary at: C:\Program Files\Android\Android Studio\jre\bin\java
โข Java version OpenJDK Runtime Environment (build 1.8.0_152-release-1136-b06)
โข All Android licenses accepted.
[โ] Android Studio (version 3.2)
โข Android Studio at C:\Program Files\Android\Android Studio
โข Flutter plugin version 31.3.1
โข Dart plugin version 181.5656
โข Java version OpenJDK Runtime Environment (build 1.8.0_152-release-1136-b06)
[โ] VS Code, 64-bit edition (version 1.30.1)
โข VS Code at C:\Program Files\Microsoft VS Code
โข Flutter extension version 2.21.1
[!] Connected device
! No devices available
! Doctor found issues in 1 category.
``` | c: crash,tool,dependency: dart,platform-windows,P2,team-tool,triaged-tool | low | Critical |
396,158,981 | flutter | Flutter google maps drag marker | Hey flutter team,
Any way to drag a marker around the map and get the location from it ? | c: new feature,waiting for PR to land (fixed),p: maps,package,team-ecosystem,P2,triaged-ecosystem | low | Critical |
396,166,893 | flutter | AnimatedSwitcher should be more customizable | Currently `AnimatedSwitcher` only allows you to play the same animation for both exit and enter, which can only be played simultaneously. If you look at the Material website, the overwhelming majority of transitions fade to white/transparent first, and then fade in the next element.
Working around both these constraints is a bit fiddly, and can be made a lot easier if these options were integrated into the constructor.
Ideally `AnimatedSwitcher` should support the following options:
- `bool simultaneous` - if `false`, plays the next `child`s animation after the previous, instead of at the same time
- `Duration {enter,exit}Duration` - specifies unique durations for entering and exiting `child`s, respectively
- `Widget {enter,exit}TransitionBuilder` - specifies unique transitions for entering and exiting `child`s, respectively | c: new feature,framework,a: animation,P2,team-framework,triaged-framework | low | Major |
396,170,657 | go | net/http: isCookieNameValid in net/http/cookie.go seems overly restrictive | Note: I attempted to post this to the golang nuts list, but my message was rejected twice for unspecified reasons.
### What version of Go are you using (`go version`)?
<pre>
go version go1.10.1 windows/amd64
</pre>
### Does this issue reproduce with the latest release?
Code in current https://github.com/golang/go/blob/master/src/net/http/cookie.go is the same as my release.
### What operating system and processor architecture are you using (`go env`)?
<details><summary><code>go env</code> Output</summary><br><pre>
set GOARCH=amd64
set GOBIN=
set GOCACHE=C:\Users\david\AppData\Local\go-build
set GOEXE=.exe
set GOHOSTARCH=amd64
set GOHOSTOS=windows
set GOOS=windows
set GOPATH=I:\golang
set GORACE=
set GOROOT=I:\Go
set GOTMPDIR=
set GOTOOLDIR=I:\Go\pkg\tool\windows_amd64
set GCCGO=gccgo
set CC=gcc
set CXX=g++
set CGO_ENABLED=1
set CGO_CFLAGS=-g -O2
set CGO_CPPFLAGS=
set CGO_CXXFLAGS=-g -O2
set CGO_FFLAGS=-g -O2
set CGO_LDFLAGS=-g -O2
set PKG_CONFIG=pkg-config
set GOGCCFLAGS=-m64 -mthreads -fno-caret-diagnostics -Qunused-arguments -fmessage-length=0 -fdebug-prefix-map=C:\Users\david\AppData\Local\Temp\go-build742879430=/tmp/go-build -gno-record-gcc-switches
</pre></details>
### What did you do?
- I make a http post call to a server ( happens to be https://www.ealing.gov.uk/site/custom_scripts/waste_collection/waste_collection.aspx )
- I receive this Set-Cookie: ```Set-Cookie: ISAWPLB{48BCE7DA-ADD0-4237-A5B8-816663CFDD23}={70F05AF6-A2D2-4861-9D98-B73CEF42E642}; HttpOnly; Path=/```
### What did you expect to see?
I expected the http package to tolerate sloppy cookie names that exist in the wild on the internet and are supported by all major browsers. This cookie name works on firefox and chrome latest. I've not tested with anything else, but given my local council is using it, and it's produced by a Microsoft application stack, I suspect it works everywhere.
### What did you see instead?
net/http/client.go attempts to handle the Set-Cookie header by calling net/http/cookie.go readSetCookies() , but fails silently, swallowing the Set-Cookie without any warning, because it deems the cookie name ```ISAWPLB{48BCE7DA-ADD0-4237-A5B8-816663CFDD23}``` invalid because it contains, as far as I can tell, ```{``` and ```}```.
This actually means that it's impossible for me to use go (without hacking net/http) to communicate properly with this web server, because the stringer on a Cookie returns "" unless the name is valid, which stops the cookie being included with outbound requests. So, even if I manually handled this badly named cookie, the http client will refuse to send it, regardless. | NeedsDecision | medium | Critical |
396,179,564 | ant-design | Unresponsive Slider when setting trigger to onAfterChange | - [x] I have searched the [issues](https://github.com/ant-design/ant-design/issues) of this repository and believe that this is not a duplicate.
### Reproduction link
[](https://z2ppnop8x.codesandbox.io/)
### Steps to reproduce
setting `options.trigger: "onAfterChange"` in `getFieldDecorator` prevents the slider from changing value
### What is expected?
The slider changes value in the same way as when options.trigger is not set. In the example that I provided I would expect the onValuesChange to fire once (at onmouseup) when options.trigger=onAfterChange.
### What is actually happening?
The use cannot change the value on the slider and onValuesChange is never called.
| Environment | Info |
|---|---|
| antd | 3.11.6 |
| React | 16.2.0 |
| System | Xubuntu 18.04 |
| Browser | Chrome 70.0.3538.77 |
<!-- generated by ant-design-issue-helper. DO NOT REMOVE --> | Inactive | low | Major |
396,184,027 | rust | MergeFunctions LLVM pass can generate invalid function calls under calling convention | Basically, the MergeFunctions LLVM pass can rewrite functions to generate calls that are not valid under the calling convention of the target, e.g. `extern "ptx-kernel"` functions should not call other `extern "ptx-kernel"` functions in NVPTX.
This is an LLVM bug, described here (thanks @nikic): https://bugs.llvm.org/show_bug.cgi?id=40232. A PR also adds a target option and a -Z flag to control MergeFunctions: https://github.com/rust-lang/rust/pull/57268.
Example: in the following Rust source, the functions `foo` and `bar` get merged by MergeFunctions:
```rust
#![crate_type = "lib"]
#![feature(abi_ptx)]
#![feature(lang_items)]
#![feature(link_llvm_intrinsics)]
#![feature(naked_functions)]
#![feature(no_core)]
#![no_core]
#[lang = "sized"]
trait Sized {}
#[lang = "copy"]
trait Copy {}
#[allow(improper_ctypes)]
extern "C" {
#[link_name = "llvm.nvvm.barrier0"]
fn syncthreads() -> ();
}
#[inline]
pub unsafe fn _syncthreads() -> () {
syncthreads()
}
#[no_mangle]
pub unsafe extern "ptx-kernel" fn foo() {
_syncthreads();
_syncthreads();
}
#[no_mangle]
pub unsafe extern "ptx-kernel" fn bar() {
_syncthreads();
_syncthreads();
}
```
to yield the incorrect PTX assembly, as the `call.uni bar` instruction is not valid since a kernel is calling another kernel (note this requires `rustc -Z merge-functions=trampolines` from the above PR):
```
//
// Generated by LLVM NVPTX Back-End
//
.version 3.2
.target sm_35
.address_size 64
// .globl bar // -- Begin function bar
// @bar
.visible .entry bar()
{
// %bb.0: // %start
bar.sync 0;
bar.sync 0;
ret;
// -- End function
}
// .globl foo // -- Begin function foo
.visible .entry foo() // @foo
{
// %bb.0:
{ // callseq 0, 0
.reg .b32 temp_param_reg;
// XXX: `call.uni bar` is not a valid call!
call.uni
bar,
(
);
} // callseq 0
ret;
// -- End function
}
```
Disabling MergeFunctions (e.g. using `rustc -Z merge-functions=disabled`) yields correct PTX assembly:
```
//
// Generated by LLVM NVPTX Back-End
//
.version 3.2
.target sm_35
.address_size 64
// .globl foo // -- Begin function foo
// @foo
.visible .entry foo()
{
// %bb.0: // %start
bar.sync 0;
bar.sync 0;
ret;
// -- End function
}
// .globl bar // -- Begin function bar
.visible .entry bar() // @bar
{
// %bb.0: // %start
bar.sync 0;
bar.sync 0;
ret;
// -- End function
}
```
P.S. Currently the default operation of MergeFunctions is to emit function aliases which are not supported by NVPTX, so controlling MergeFunctions via the `merge-functions` flag is necessary to generate any of the PTX assembly above.
## Meta
I'm on a patched rustc so this may not be so helpful, but here it is anyway:
`rustc --version --verbose`:
rustc 1.33.0-nightly (fb86d604b 2018-12-27)
binary: rustc
commit-hash: fb86d604bf65c3becd16180b56267a329cf268d5
commit-date: 2018-12-27
host: x86_64-unknown-linux-gnu
release: 1.33.0-nightly
LLVM version: 8.0 | A-LLVM,P-medium,T-compiler,C-bug | low | Critical |
396,191,003 | TypeScript | Add ReadonlyDate | We have `ReadonlyArray`, `ReadonlyMap`, `ReadonlySet`, so we need `ReadonlyDate` :) | Suggestion,Domain: lib.d.ts,Awaiting More Feedback | low | Major |
396,227,773 | flutter | Support home and lock screen widgets | Both Android and iOS have widgets that can be used outside of apps. On Android they are home screen widgets, and on iOS there are lock screen/notification center widgets. Would it be possible to create them using Flutter widgets? | c: new feature,platform-android,platform-ios,framework,engine,customer: crowd,P3,team-engine,triaged-engine | high | Critical |
396,236,878 | flutter | Baseline proper in icon widget to better align some icons for aesthetics | Some icons look better together when one is slightly offset vertically.
<img src="https://user-images.githubusercontent.com/20849728/50733546-9e253e00-11e3-11e9-8319-4f885d599106.png" width=400 />
The thumb icons in my music application are a great example of this. Right now, there's no easy way to align the icons so the bottoms of the hands are aligned.
It'd be great if we had some sort of "icon baseline" property that we could use to align our icons better.
It's also important for the hitboxes of the icons to still be vertically aligned though. | c: new feature,framework,f: material design,P2,team-design,triaged-design | low | Major |
396,240,665 | pytorch | Implicit conversion error in caffe2 | Hello,
While compiling generated sources(caffe2.pb.cc) from caffe2.proto we are getting the implicit conversion error
protobuf version=v3.5.2
compiler:
CC=aarch64-linux-android-clang \
CXX=aarch64-linux-android-clang++
```
/home/armnn1/armnn-devenv/armnn/src/armnnCaff2Parser/proto/caffe2.pb.cc:2269:9: error: implicit conversion changes signedness: '::google::protobuf::int32' (aka 'int') to
'google::protobuf::uint32' (aka 'unsigned int') [-Werror,-Wsign-conversion]
static_cast< ::google::protobuf::int32>(
^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
/home/armnn1/armnn-devenv/armnn/src/armnnCaff2Parser/proto/caffe2.pb.cc:2282:9: error: implicit conversion changes signedness: '::google::protobuf::int32' (aka 'int') to
'google::protobuf::uint32' (aka 'unsigned int') [-Werror,-Wsign-conversion]
static_cast< ::google::protobuf::int32>(
^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
/home/armnn1/armnn-devenv/armnn/src/armnnCaff2Parser/proto/caffe2.pb.cc:2326:9: error: implicit conversion changes signedness: '::google::protobuf::int32' (aka 'int') to
'google::protobuf::uint32' (aka 'unsigned int') [-Werror,-Wsign-conversion]
static_cast< ::google::protobuf::int32>(
^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
/home/armnn1/armnn-devenv/armnn/src/armnnCaff2Parser/proto/caffe2.pb.cc:2339:9: error: implicit conversion changes signedness: '::google::protobuf::int32' (aka 'int') to
'google::protobuf::uint32' (aka 'unsigned int') [-Werror,-Wsign-conversion]
static_cast< ::google::protobuf::int32>(
^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
/home/armnn1/armnn-devenv/armnn/src/armnnCaff2Parser/proto/caffe2.pb.cc:3017:9: error: implicit conversion changes signedness: '::google::protobuf::int32' (aka 'int') to
'google::protobuf::uint32' (aka 'unsigned int') [-Werror,-Wsign-conversion]
static_cast< ::google::protobuf::int32>(
^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
```
Thank you,
| caffe2 | low | Critical |
396,249,376 | go | cmd/vet: govet sometimes wrong about whether a context gets cancelled | ### What version of Go are you using (`go version`)?
Not sure, it's a toolchain. Most likely 1.11.
### Does this issue reproduce with the latest release?
Yes, as of 1.12beta.
### What operating system and processor architecture are you using (`go env`)?
No idea, govet runs remotely on a language server.
### What did you do?
```Go
ctx, cancel := context.WithCancel(context.Background())
defer cancel()
if true {
// This makes govet think that the child context never gets cancelled,
// when, in fact, it is cancelled by the parent's cancel().
ctx, _ = context.WithTimeout(ctx, time.Second)
}
```
### What did you expect to see?
govet should not claim that the child context leaks. (If it's a style question, it should be in the linter, not govet.)
### What did you see instead?
govet incorrectly thinks the child context does not get cancelled. | help wanted,NeedsInvestigation,Analysis | low | Major |
396,252,617 | rust | [NLL] Bad higher ranked subtype error | With the removal of the leak check the MIR type checker is now responsible for reporting higher-ranked lifetime errors in full NLL mode. The error messages are not currently very helpful, since they weren't user visible until now.
The following code ([play](https://play.rust-lang.org/?version=nightly&mode=debug&edition=2018&gist=d5c8f78908722b83ba0610188ad6b9ef)):
```rust
#![feature(nll)]
fn main() {
let x: fn(&'static ()) = |_| {};
let y: for<'a> fn(&'a ()) = x;
}
```
gives the following output
```text
error: higher-ranked subtype error
--> <source>:19:12
|
19 | let x: fn(&'static ()) = |_| {};
| ^^^^^^^^^^^^^^^
error: aborting due to previous error
Compiler returned: 1
```
In migrate mode or with AST borrowck the error is much clearer:
```text
error[E0308]: mismatched types
--> <source>:20:33
|
20 | let y: for<'a> fn(&'a ()) = x;
| ^ one type is more general than the other
|
= note: expected type `for<'a> fn(&'a ())`
found type `fn(&'static ())`
error: aborting due to previous error
For more information about this error, try `rustc --explain E0308`.
Compiler returned: 1
```
cc @rust-lang/wg-compiler-nll | A-diagnostics,P-medium,T-compiler,A-NLL,NLL-diagnostics,A-higher-ranked | medium | Critical |
396,257,621 | godot | Changing a value by dragging floods the output window with "Set <property_name>" messages | **Godot version:**
3.1 Alpha 5
**OS/device including version:**
Kubuntu 18.10
**Issue description:**
Changing a number value in the property editor by dragging (using the mouse) leaves a "Set <property_name>" message on every frame
It would be nice to have a way to turn it off or only leave a message _after_ changing the value (letting go of the mouse button)
**Steps to reproduce:**
* On any project, Click on a node (With numbered values, like a node2d) to reveal its properties.
* Click and drag on a numbered value to change its value.
* Watch how the output window starts outputting a lot of the same message.
**Minimal reproduction project:**
(None needed)
In my opinion it's quite annoying because it makes scrolling through the output window unnecessarily harder.
Edit: output window, not the debug window | enhancement,topic:editor,confirmed | low | Critical |
396,265,017 | rust | DerefMut borrow method call is too long with Deref arguments | Currently with Rust 2018, this code does not compile.
```rust
fn main() {
let mut v = vec![1, 2, 3];
v.swap(0, v.len() - 1);
assert_eq!(v, &[3, 2, 1]);
}
```
```
error[E0502]: cannot borrow `v` as immutable because it is also borrowed as mutable
|
3 | v.swap(0, v.len() - 1);
| - ---- ^ immutable borrow occurs here
| | |
| | mutable borrow later used by call
| mutable borrow occurs here
```
It seems that the compiler desugars the method call like this:
```rust
let v0 = &*v;
let v1 = &mut *v;
let arg0 = 0;
let arg1 = v0.len() - 1; // v1 is still alive here
v1.swap(arg0, arg1);
```
But I think the `DerefMut` should be deferred, or use only `DerefMut` reference without any `Deref`.
```rust
let v0 = &*v;
let arg0 = 0;
let arg1 = v0.len() - 1; // no mutable borrows here
let v1 = &mut *v;
v1.swap(arg0, arg1);
```
```rust
let v0 = &mut *v;
let arg0 = 0;
let arg1 = v0.len() - 1;
v0.swap(arg0, arg1);
``` | P-medium,T-compiler,A-NLL,NLL-complete | low | Critical |
396,267,448 | rust | Inappropriate `warning: unreachable expression` in `println!` | `return` expression in call params of `println!` macro causes inappropriate warning message.
I tried this code (ref: [Playground](https://play.rust-lang.org/?version=stable&mode=debug&edition=2018&gist=1c8219aa795635c0717ba3e5a2a10ad3)):
```rust
fn func1() {
assert_eq!((), return ());
}
fn func2() {
println!("{}", return ());
}
fn main() {
func1();
func2();
}
```
I expected to print `^^^` against an entire statement:
```text
warning: unreachable expression
--> src/main.rs:2:5
|
2 | assert_eq!((), return ());
| ^^^^^^^^^^^^^^^^^^^^^^^^^^
|
= note: #[warn(unreachable_code)] on by default
= note: this error originates in a macro outside of the current crate (in Nightly builds, run with -Z external-macro-backtrace for more info)
warning: unreachable expression
--> src/main.rs:2:5
|
2 | assert_eq!((), return ());
| ^^^^^^^^^^^^^^^^^^^^^^^^^^
|
= note: this error originates in a macro outside of the current crate (in Nightly builds, run with -Z external-macro-backtrace for more info)
warning: unreachable expression
--> src/main.rs:6:20
|
6 | println!("{}", return ());
| ^^^^^^^^^^^^^^^^^^^^^^^^^^
```
But, it was printed against an expression instead of a sentence:
```text
warning: unreachable expression
--> src/main.rs:2:5
|
2 | assert_eq!((), return ());
| ^^^^^^^^^^^^^^^^^^^^^^^^^^
|
= note: #[warn(unreachable_code)] on by default
= note: this error originates in a macro outside of the current crate (in Nightly builds, run with -Z external-macro-backtrace for more info)
warning: unreachable expression
--> src/main.rs:2:5
|
2 | assert_eq!((), return ());
| ^^^^^^^^^^^^^^^^^^^^^^^^^^
|
= note: this error originates in a macro outside of the current crate (in Nightly builds, run with -Z external-macro-backtrace for more info)
warning: unreachable expression
--> src/main.rs:6:20
|
6 | println!("{}", return ());
| ^^^^^^^^^
```
## Meta
`rustc --version --verbose`: 1.31.1
| C-enhancement,A-lints,A-diagnostics,A-macros,T-compiler | low | Critical |
396,285,940 | neovim | Turn neovim into $PAGER | Hello,
I'm author of [page](https://github.com/I60R/page) - a neovim's client similar to neovim-remote but rather focused on redirecting text from one :term buffer and reading it in another. It was created with intention to merge `less` functionality into `neovim` and provide more integrated paging for better terminal experience. The goal achieved pretty well, although currently `page` has some severe limitations that only can be resolved on `neovim` side.
The limitations are:
### 1. Scrollback maximum on :term buffer
`page` uses :term buffer to render ANSI escape sequences. But in neovim :term buffer scrollback is limited to 100000 lines and while it's enough for terminal purposes for pager it's not sufficient. When this maximum is over `page` loses possibly relevant text with some strange effects I60R/page#7. This is the worst of listed issues and having option for unlimited scrollback would be really valuable to resolve it.
### 2. MANPAGER='page -t man' not works (-t provides hint for ftplugin)
Neovim's `man` filetype fails on :term and read-only buffers:
* `page` sets `nomodifiable` and then `ft=man` which produces `E21: Cannot make changes, \'modifiable\' is off: silent keepjumps 1delete _`
* without `nomodifiable` it produces `E474: Invalid argument: buftype=nofile`
Currently I recommend to use workaround with copy-pasting into non-:term buffer but looks like only some small tweaks required in `man.vim` to resolve this.
### 3. Lack of on-demand text fetching
E.g `less` pager blocks its stdin and reads from it only when required. This prevents from lags and redundant large computations e.g. when reading output of `journalctl` (which is pretty common use case). Current solution is to manually fetch lines on `:Page` command which also should be explicitly enabled with `-q <number_of_lines>` argument.
But it seems to be possible to implement auto-fetching on scrolling by utilizing of `CursorMoved` autocommand. I would like to use it instead, however without auto-fetch on searching and probably in other contexts it would be surprising and inconvenient for users.
Maybe `SearchFound` and `SearchNotFound` autocommands or something like that would help to mimic `less` behavior better? I'm not sure if this is a good idea through, since it might be too complex to implement; it's already possible to override default <number_of_lines> using `:Page <count>` command, so it may be not worth it...
---
Could you collaborate on that? | enhancement,terminal,input,pager | low | Major |
396,291,334 | flutter | Hero should allow specifying a curve and reverseCurve | Currently in `heroes.dart`:
```dart
Animation<double> get animation {
return CurvedAnimation(
parent: (type == HeroFlightDirection.push) ? toRoute.animation : fromRoute.animation,
curve: Curves.fastOutSlowIn,
);
}
```
`Curves.fastOutSlowIn` looks decent for an entry curve, however when the hero animation is played in reverse when going to the previous route, you get something like the following:
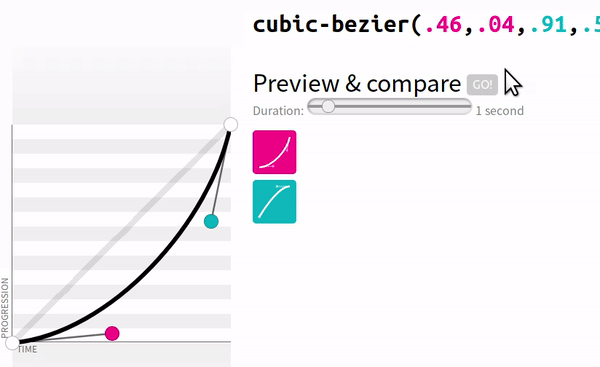
This could be solved by checking `HeroFlightDirection.push/pull` and flipping the curve if necessary, but IMO it would make more sense to allow the user to specify `curve` and `reverseCurve` like most of the other animation APIs we have. `curve` would naturally be flipped when going backwards if `reverseCurve` isn't specified.
When I was working on improving the animations of `CupertinoPageRoute`, the Hero animation of the title becoming the back button was the one thing I couldn't alter, since there isn't an API to specify a reverse curve. Example (watch the Colors title):
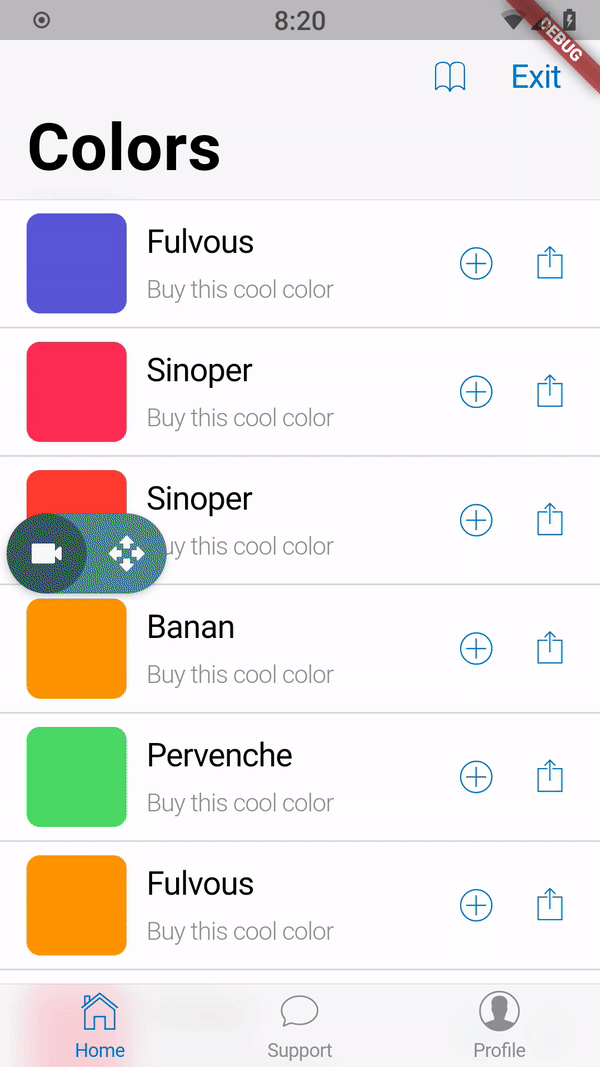 | c: new feature,framework,f: material design,P2,team-design,triaged-design | low | Major |
396,298,106 | flutter | Tizen platform support | Hi, any plans for [tizen](https://www.tizen.org) support? | c: new feature,engine,customer: crowd,P3,team-engine,triaged-engine | high | Critical |
396,306,881 | rust | Tracking issue for duration_constants | Implemented in https://github.com/rust-lang/rust/pull/57375/
> This will make working with durations more ergonomic. Compare:
>
> ```rust
> // Convenient, but deprecated function.
> thread::sleep_ms(2000);
>
> // The current canonical way to sleep for two seconds.
> thread::sleep(Duration::from_secs(2));
>
> // Sleeping using one of the new constants.
> thread::sleep(2 * SECOND);
> ```
```rust
impl Duration {
pub const SECOND: Duration = Duration::from_secs(1);
pub const MILLISECOND: Duration = Duration::from_millis(1);
pub const MICROSECOND: Duration = Duration::from_micros(1);
pub const NANOSECOND: Duration = Duration::from_nanos(1);
pub const MAX: Duration = ...;
} | T-libs-api,B-unstable,C-tracking-issue,Libs-Tracked,A-time | high | Critical |
396,310,226 | TypeScript | Readonly properties type narrowing doesn't flow into inner function scopes | <!-- ๐จ STOP ๐จ ๐ฆ๐ง๐ข๐ฃ ๐จ ๐บ๐ป๐ถ๐ท ๐จ
Half of all issues filed here are duplicates, answered in the FAQ, or not appropriate for the bug tracker. Even if you think you've found a *bug*, please read the FAQ first, especially the Common "Bugs" That Aren't Bugs section!
Please help us by doing the following steps before logging an issue:
* Search: https://github.com/Microsoft/TypeScript/search?type=Issues
* Read the FAQ: https://github.com/Microsoft/TypeScript/wiki/FAQ
Please fill in the *entire* template below.
-->
<!-- Please try to reproduce the issue with `typescript@next`. It may have already been fixed. -->
**TypeScript Version:** 3.3.0-dev.20190105
<!-- Search terms you tried before logging this (so others can find this issue more easily) -->
**Search Terms:** Readonly, strictNullChecks, optional, inner function
**Code**
Using `strictNullChecks: true`:
```ts
interface Thing {
foo?: (a: number) => boolean;
}
const t: Readonly<Thing> = {
foo: (a) => true,
};
if (t.foo !== undefined) {
const bar = () => {
t.foo(5);
}
}
```
**Expected behavior:**
๐ Compile
**Actual behavior:**
On the expression `t.foo(5);` the following error:
```
Cannot invoke an object which is possibly 'undefined'.
(property) foo?: ((a: number) => boolean) | undefined
```
**Playground Link:** https://www.typescriptlang.org/play/index.html#src=interface%20Thing%20%7B%0D%0A%20%20%20%20foo%3F%3A%20(a%3A%20number)%20%3D%3E%20boolean%3B%0D%0A%7D%0D%0A%0D%0Aconst%20t%3A%20Readonly%3CThing%3E%20%3D%20%7B%0D%0A%20%20%20%20foo%3A%20(a)%20%3D%3E%20true%2C%0D%0A%7D%3B%0D%0A%0D%0Aif%20(t.foo%20!%3D%3D%20undefined)%20%7B%0D%0A%20%20%20%20const%20bar%20%3D%20()%20%3D%3E%20%7B%0D%0A%20%20%20%20%20%20%20%20t.foo(5)%3B%0D%0A%20%20%20%20%7D%0D%0A%7D%0D%0A%0D%0A
**Related Issues:**
* Possibly a duplicate https://github.com/Microsoft/TypeScript/issues/22137, but I wasn't sure.
* Following the theory of this issue, this should be seen as a bug: https://github.com/Microsoft/TypeScript/issues/10927.
| Suggestion,Experience Enhancement,Domain: Control Flow | low | Critical |
396,313,039 | rust | Suggest code to use enum type in return type when accidentally using variant instance | When encountering an enum variant instance in a return type, make a better effort to suggest appropriate code.
Right now, if you accidentally write `Some(Span)` when you meant `Option<Span>`, we mention the existence of the enum, but we don't suggest code:
```
error[E0573]: expected type, found variant `Some`
--> src/librustc_typeck/check/mod.rs:5198:10
|
5198 | ) -> Some(Span) {
| ^^^^^^^^^^ not a type
|
= help: there is an enum variant `rustc::middle::cstore::LibSource::Some`, try using `rustc::middle::cstore::LibSource`?
= help: there is an enum variant `rustc::session::config::Passes::Some`, try using `rustc::session::config::Passes`?
= help: there is an enum variant `std::prelude::v1::Option::Some`, try using `std::prelude::v1::Option`?
= help: there is an enum variant `std::prelude::v1::Some`, try using `std::prelude::v1`?
```
It'd be nice if we could suggest the appropriate code. | C-enhancement,A-diagnostics,T-compiler,A-suggestion-diagnostics,D-invalid-suggestion | low | Critical |
396,340,261 | react | Nested portals should be discoverable | This is more about a bridge between actual DOM Tree and React Tree.
**Do you want to request a *feature* or report a *bug*?**
feature
**What is the current behavior?**
You can _portal_ a part of your rendering tree to another place in Dom Tree, and React would handle events, including events on Capture Phase like there were no portals - events could dive through all the _react_ parents, and bubble up through all the _react_ parents.
This is quite useful, as long as portal is an implementation detail, but useful only for normal events; there are more cases around it.
**What is the expected behavior?**
It's better to explain it by example
- you have a Modal Dialog and it uses a Focus Lock, ie focus could not leave it.
- inside Modal you have a Custom Select, with Dropdown menu rendered via a portal.
- you could not use it, as long as from DOM prospective `ModalNode.contains(DropDownNode)` is always false, and Focus Lock will prevent focusing.
It's a real issue - https://github.com/reach/reach-ui/issues/83, https://github.com/theKashey/react-focus-lock/issues/19.
Proposed solution:
- `containsNode(domNode):boolean` - _React-aware_ version of DOM API `node.contains(anotherNode)`.
- `getHostNodes():Nodes[]` - returns a list of all root nodes inside "current component" including direct children and portals. Similar to `ReactDom.findDomNode`, and (proposed)refs attached to React.Fragment. It just finds all nodes "you are consists of". As a result you will be able to `tab` from one `piece` of you to another, making focus management independed of implementation details.
Cons:
- requires Component to access fiber, DOM node to access fiber thought node, or an new `hook` to do it in a functional way.
- does twice dreadfull things than deprecated `findDomNode`
- usage scope is very narrow.
Pros:
- _my_ use case requires _momentum_ access to a rendered tree, and does not suffer async stuff as `findDomNode`, where underlaying node might not be yet created. Stuff like "_does something `containsNode` right now_", or _"`getHostNodes` I consist from right now"_ are sync, and the question asked about actual DOM tree structure.
Example using [react-dom-reflection](https://github.com/theKashey/react-dom-reflection), which implements required API - https://codesandbox.io/s/1or60v506l
**Which versions of React, and which browser / OS are affected by this issue? Did this work in previous versions of React?**
Never worked | Type: Feature Request | medium | Critical |
396,354,045 | vscode | Emmet toggle comment not working correctly for jsx | - VSCode Version: 1.30.1
- OS Version: win10 v1803
This issue occurs for both js & jsx extension files
Steps to Reproduce:
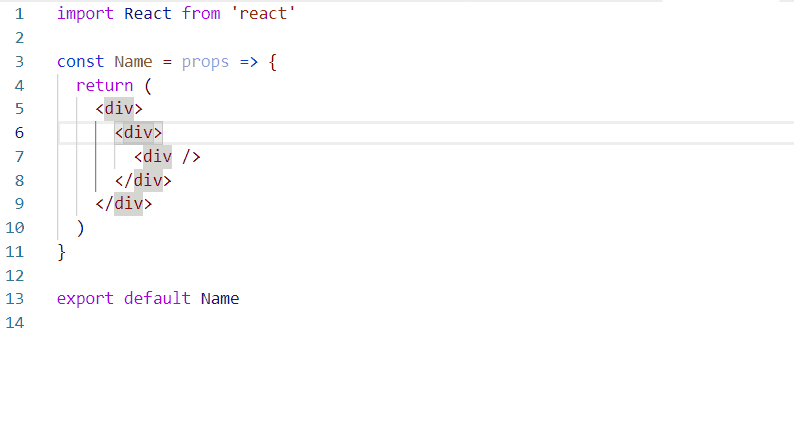
<!-- Launch with `code --disable-extensions` to check. -->
Does this issue occur when all extensions are disabled?: Yes
| bug,emmet,emmet-parse | low | Critical |
396,383,376 | rust | Lifetime elision applies differently in traits and impls with explicit self type | A specific function signature works in trait decl, but not in the impl:
```rust
trait Silly {
// OK
fn id(self: &Self) -> &Self;
}
impl<T> Silly for T {
// error[E0106]: missing lifetime specifier
fn id(self: &T) -> &T { self}
// ^ help: consider giving it a 'static lifetime: `&'static`
}
```
[playground](https://play.rust-lang.org/?version=stable&mode=debug&edition=2018&gist=2e0094386c365b2e0e7d88911432ddf8) | A-lifetimes,T-compiler,A-inference,C-bug | low | Critical |
396,389,107 | vscode | autoclosing markdown preview when closing md file | Issue Type: <b>Feature Request</b>
When editing markdown file, it is quite convenient to have the preview in a aplit view. However, when I switch to another opened file (or closing the md file), the preview of markdown and the split is still there. This is annoying because I can only use half of the window for the new file.
VS Code version: Code 1.30.1 (dea8705087adb1b5e5ae1d9123278e178656186a, 2018-12-18T18:05:00.654Z)
OS version: Darwin x64 18.2.0
<!-- generated by issue reporter --> | feature-request,markdown | medium | Major |
396,441,613 | nvm | Using latest node subversion of any version | Possible feature request if not already implemented.
If I want to use the latest node 6 version (6.16.0 at the moment), is it possible to install it using nvm install 6.x ? I couldn't find it in the documentation and tried doing:
```
nvm install 6.16.0
nvm use 6.x
```
which throws a
```
N/A: version "6.x -> N/A" is not yet installed.
You need to run "nvm install 6.x" to install it before using it.
``` | installing node | low | Minor |
396,453,088 | kubernetes | Support arbitrary subresources for custom resources | Currently, custom resources support only `status` and `scale` subresources. The only way to use custom subresources with CRs is to use an aggregated apiserver.
Given that using CRDs is comparatively easier than setting up an aggregated apiserver, there have been requests [[1](https://github.com/kubernetes/enhancements/issues/571#issuecomment-450404761)][[2](https://github.com/kubernetes/apiextensions-apiserver/issues/33)] for supporting arbitrary subresources with CRDs.
I don't think we intended to support this or have this on our roadmap in the near future. But we don't have a formal consensus on this yet. I created this issue to have a discussion and gather consensus.
/sig api-machinery
/area custom-resources
/kind feature
/cc @sttts @liggitt @deads2k @lavalamp @mbohlool
| priority/backlog,sig/api-machinery,kind/feature,area/custom-resources,lifecycle/frozen | high | Critical |
396,466,237 | TypeScript | Improve Type guards to correctly work with for-of loops | <!-- ๐จ STOP ๐จ ๐ฆ๐ง๐ข๐ฃ ๐จ ๐บ๐ป๐ถ๐ท ๐จ
Half of all issues filed here are duplicates, answered in the FAQ, or not appropriate for the bug tracker. Please read the FAQ first, especially the "Common Feature Requests" section.
-->
## Search Terms
for-of, Type Guard
<!-- List of keywords you searched for before creating this issue. Write them down here so that others can find this suggestion more easily -->
## Suggestion
At the moment a paradigm of call-Parameter-Testing I apply works well in case of one parameter, but if I want to shorten the testing of multiple parameters using a for-of loop the type assertion fails.
<!-- A summary of what you'd like to see added or changed -->
## Use Cases
Correct type guarding of multiple parameters which are supposed to be the same type but to be handled differently
<!--
What do you want to use this for?
What shortcomings exist with current approaches?
-->
## Examples
```ts
type generalObj<T> = {
value: T,
};
type numObj = generalObj<number>
const isNumObj = (testObj: generalObj<any>): testObj is numObj => {
//some logic here...
return true;
}
const numConsumer = (param: numObj) => param;
const shouldWork = (shouldBeNumObj: generalObj<number | string>) => {
for (const c of [shouldBeNumObj]) {
if (!isNumObj(c)) {
return console.error('no numObj')
}
}
// error here despite the right type ensured
numConsumer(shouldBeNumObj);
}
const doesWork = (shouldBeNumObj: generalObj<number | string>) => {
if (!isNumObj(shouldBeNumObj)) {
return console.error('no numObj')
}
numConsumer(shouldBeNumObj);
}
const shouldWork2 = (shouldBeNumObj: Array<generalObj<number | string>>) => {
for (const c of shouldBeNumObj) {
if (!isNumObj(c)) {
return console.error('no numObj')
}
}
numConsumer(shouldBeNumObj[0]);
}
const baseTypeNumConsumer = (param: number) => param;
// curtesy of @nmain
const shouldWork3 = (shouldBeNum: string | number) => {
for (const v of [shouldBeNum]) {
if (typeof v !== "number") {
return;
}
}
baseTypeNumConsumer(shouldBeNum); // compiler error, but should be valid
}
const stringConsumer = (param: generalObj<string>) => param;
const couldBreakCode = (anyObjS: Array<generalObj<any>>) => {
for (const c of anyObjS) {
if (!isNumObj(c)) {
return console.error('no numObj')
}
}
stringConsumer(anyObjS[0])
}
const becauseThisFails = (anyObj: generalObj<any>) => {
if (!isNumObj(anyObj)) {
return console.error('no numObj')
}
stringConsumer(anyObj);
}
```
<!-- Show how this would be used and what the behavior would be -->
## Checklist
My suggestion meets these guidelines:
* [ ] This wouldn't be a breaking change in existing TypeScript/JavaScript code
- Could break compilation of already error-prone code where Type testing was disabled with `any `
* [x] This wouldn't change the runtime behavior of existing JavaScript code
* [x] This could be implemented without emitting different JS based on the types of the expressions
* [x] This isn't a runtime feature (e.g. library functionality, non-ECMAScript syntax with JavaScript output, etc.)
* [x] This feature would agree with the rest of [TypeScript's Design Goals](https://github.com/Microsoft/TypeScript/wiki/TypeScript-Design-Goals).
| Bug,Domain: Control Flow | low | Critical |
396,495,621 | vscode | [html] code complete for href anchors | <!-- Please search existing issues to avoid creating duplicates. -->
<!-- Also please test using the latest insiders build to make sure your issue has not already been fixed: https://code.visualstudio.com/insiders/ -->
<!-- Use Help > Report Issue to prefill these. -->
- VSCode Version: Version: 1.30.1 (user setup)
Commit: dea8705087adb1b5e5ae1d9123278e178656186a
Date: 2018-12-18T18:12:07.165Z
Electron: 2.0.12
Chrome: 61.0.3163.100
Node.js: 8.9.3
V8: 6.1.534.41
- OS Version: Windows_NT x64 10.0.17134
Steps to Reproduce:
1. Create html file and some elements with their own id like so: `<div id="TEXTHERE">`
2. Create an `<a>` tag with href like this: `<a href="#TEXTHERE>content</a>`
3. You will see no autocompletion for the href. If you reload VSCode fresh the autocompletion works for a few secs (via word autocompletion) but stops to work when VSCode has completed all startup processes.
Use-case: You have a static HTML file with a lot of elements with IDs and want to check while typing in an anchor link if that ID exists.
I tried three different autocompletion plugins, none of them could give me this functionality.
This works flawlessly in Atom, though.
<!-- Launch with `code --disable-extensions` to check. -->
Does this issue occur when all extensions are disabled?: **Yes** | feature-request,html | low | Minor |
396,511,297 | flutter | Consider make flutter/engine truly platform-agnostic/portable | Hello folks!
Flutter Engine claims to be truly platform agnostic, self-contained and portable. In reality we are not there yet. There are a bunch of code in the engine repo which is platform-specific like the ones here: https://github.com/flutter/engine/tree/master/fml/platform
We are porting flutter to a very restrict and secure ARM device. So far we are having success except that flutter on linux is requiring `timerfd_create` syscall in order to create timers but, for security reasons, the device doesn't allow that and that syscall isn't implemented.
That is a clear case where the engine is embracing platform specific code which should be on the embeder or PAL.
We will make that to work by emulating that syscall all over the place, which is far from desirable.
It would be good if those platform-specific code got moved elsewhere and that way the engine would became what it is meant to be... A clean and slick engine which when initialised takes as parameter all the required platform-specific implementations. That way we open flutter to even more target platforms.
We are pretty new on flutter and we're learning as we walk every step on the port process. If there is something that achieve what I'm asking already in place, please forgive the noobish and we would appreciate if you can point us to the right direction.
Thanks! | c: new feature,engine,dependency: dart,e: embedder,P3,team-engine,triaged-engine | medium | Major |
396,518,204 | flutter | Deep-linking support for Navigator | Is there a particular way to make Flutter's Navigator have a specific navigation history if you for example want to send in a new navigation history based on an Android notification?
For example, start the `FlutterView` at `[Main, List, Detail[someId]]`? | c: new feature,framework,d: api docs,f: routes,customer: crowd,P2,team-framework,triaged-framework | low | Critical |
396,577,090 | rust | Suggestion for pub(crate) and reexports are tricked | First [reported upstream](https://github.com/rust-lang/cargo/issues/6465#issuecomment-448836441), this code:
```rust
#![warn(unreachable_pub)]
#![deny(unused_imports)]
pub mod core {
pub mod compiler {
mod context {
mod compilation_files {
pub struct Metadata;
pub struct CompilationFiles;
impl CompilationFiles {
pub fn metadata() -> Metadata { Metadata }
}
}
use self::compilation_files::CompilationFiles;
pub use self::compilation_files::Metadata; // Suggests pub(crate)
// but that causes unused_imports to complain.
pub struct Context;
impl Context {
pub fn files() -> CompilationFiles { CompilationFiles }
}
}
pub use self::context::Context;
}
}
```
[generates the warning](https://play.rust-lang.org/?version=nightly&mode=debug&edition=2018&gist=4202a0227a013abc063afcb5d881a3e3)
```
warning: unreachable `pub` item
--> src/lib.rs:15:13
|
15 | pub use self::compilation_files::Metadata; // Suggests pub(crate)
| ---^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
| |
| help: consider restricting its visibility: `pub(crate)`
|
note: lint level defined here
--> src/lib.rs:1:9
|
1 | #![warn(unreachable_pub)]
| ^^^^^^^^^^^^^^^
= help: or consider exporting it for use by other crates
warning: unreachable `pub` item
--> src/lib.rs:15:21
|
15 | pub use self::compilation_files::Metadata; // Suggests pub(crate)
| --- ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
| |
| help: consider restricting its visibility: `pub(crate)`
|
= help: or consider exporting it for use by other crates
```
but the suggested fix doesn't compile! | T-compiler,C-bug,A-suggestion-diagnostics,D-invalid-suggestion,L-unreachable_pub | low | Critical |
396,597,085 | flutter | image_picker plugin should allow generation of thumbnail images | Since the image_picker returns the full resolution images and flutter doesn't automagicly shrink images based on the rendered size, it is easy to trigger OOM/memory pressure when using the returned images. (See https://github.com/flutter/flutter/issues/21571). Regardless of the decisions we make regarding OOM handling for images, it would be nice if the image_picker could also generate much smaller thumbnails to make it easy to avoid loading the full size images.
Example:
```
ImageWithThumbnail imageWithThumbnail = await ImagePicker.pickImageWithThumbnail(source: ImageSource.camera, thumbnailSize: Size(100, 100));
class ImageWithThumbnail {
File get image
File get thumbnail
}
| c: new feature,p: image_picker,package,c: proposal,team-ecosystem,P3,triaged-ecosystem | medium | Major |
396,598,075 | vscode | Find usages (find all file references / find all folder references) | If you search the issues here on GitHub and on StackOverflow, you'll find that there is a ton of fragmentation around this feature for a few reasons:
- Everyone is referring to different subsets of the feature
- Everyone is calling it by different names (users coming from WebStorm will refer to it as "Find Usages")
- VSCode actually already has this partially implemented, but it's hidden and not documented well.
This feature refers to three subsets of finding references:
**1. Find all references of a symbol**
VSCode has this already.
**2. Find all references of a file**
VSCode has this already, but few know about it, and the process for getting to it is unnecessary. Currently you need to use the "find all references" command on an import path that includes the file.
This is a very useful feature, and should be made available in the file explorer, for a file's context menu when right clicking it, [as explained here](https://github.com/Microsoft/vscode/issues/66150). Additionally, there should be a command, `References: Find All References of File` as [explained here](https://github.com/Microsoft/vscode/issues/41681).
**3. Find all references of a folder**
VSCode does not yet have this feature. When using this on a folder (made available in the context menu), it should show the references for all files inside that folder. A command might be useful here as well (`References: Find All References of Folder`).
**Note:**
I've already submitted [a feature request](https://github.com/Microsoft/vscode/issues/52845) mentioning this in the past, which was closed and suggested as an extension. However, it looks like I wasn't clear on what my request was, because VSCode has since implemented a lot of this functionality. And it's also clear that there's a lot of confusion around this among VSCode users.
| feature-request,editor-symbols | medium | Critical |
396,607,348 | TypeScript | Unexpected implicit any for return value of Array<T>.indexOf |
<!-- ๐จ STOP ๐จ ๐ฆ๐ง๐ข๐ฃ ๐จ ๐บ๐ป๐ถ๐ท ๐จ
Half of all issues filed here are duplicates, answered in the FAQ, or not appropriate for the bug tracker. Even if you think you've found a *bug*, please read the FAQ first, especially the Common "Bugs" That Aren't Bugs section!
Please help us by doing the following steps before logging an issue:
* Search: https://github.com/Microsoft/TypeScript/search?type=Issues
* Read the FAQ: https://github.com/Microsoft/TypeScript/wiki/FAQ
Please fill in the *entire* template below.
-->
<!-- Please try to reproduce the issue with `typescript@next`. It may have already been fixed. -->
**TypeScript Version:** 3.3.0-dev.20190105
<!-- Search terms you tried before logging this (so others can find this issue more easily) -->
**Search Terms:** evolving array type, indexOf
**Code**
```js
const arr = [];
arr.push(1);
while (true) {
const index = arr.indexOf(1);
arr[index] = 1;
}
```
**Expected behavior:**
No errors.
**Actual behavior:**
```
'index' implicitly has type 'any' because it does not have a type annotation and is referenced directly or indirectly in its own initializer.
```
**Playground Link:** [(turn on `noImplicitAny`)](http://www.typescriptlang.org/play/#src=const%20arr%20%3D%20%5B%5D%3B%0A%0Aarr.push(1)%3B%0Aarr%3B%0A%0Awhile%20(true)%20%7B%0A%20%20%20%20const%20index%20%3D%20arr.indexOf(1)%3B%0A%20%20%20%20arr%5Bindex%5D%20%3D%201%3B%0A%7D)
**Related Issues:** #19955
**In the wild:** https://github.com/GoogleChrome/lighthouse/pull/6874#discussion_r244243029. Note @brendankenny's educated guess on [what is going on](https://github.com/GoogleChrome/lighthouse/pull/6874#discussion_r245186223). | Bug,Domain: Control Flow | low | Critical |
396,617,344 | rust | perform macro-expansion on doctests before checking for `fn main` | As discussed in https://github.com/rust-lang/rust/pull/57019, i'd like to refactor the doctest parsing/execution code in rustdoc so that it checks for `fn main` and `extern crate` *during* compilation, not before. This allows us to take advantage of macro expansion and a proper parsing step to find multi-line attributes or `extern crate` statements with the `#[macro_use]` on a separate line.
I believe it's possible to break up the compilation steps in `test::run_test` like in `core::run_core`, except this time we'd stop after `phase_2` and inspect the crate for our desired items. If the test doesn't have a `fn main` or the right `extern crate` statement, then we can modify the crate in-place (or attempt to splice in the right text in the original text and run compilation again) before continuing. I haven't tried it, but i'd like to sketch it out soon. | T-rustdoc,C-feature-request,A-doctests | low | Minor |
396,625,583 | TypeScript | Javascript, Nodejs: Go to definition for required file does not work if required file does not contain exports. | a.js
```js
require('./b.js'); // Go to definion does not work here. But 'Find all references' does work.
require('./c.js'); // Go to definion works here.
```
b.js
```js
// no exports or module.exports assigns here, just some side effects.
```
c.js
```
exports.a = 42;
```
VSCode 1.30.1.
Linux 4.18 x64..
> Does this issue occur when all extensions are disabled?: Yes/No
Yes.
| Bug,Domain: TSServer,Domain: Symbol Navigation | low | Minor |
396,627,231 | TypeScript | Show unused public properties and methods | It would be nice to have unused detection expanded, capable of detecting unused methods, exports, etc.
**Code Example**
_Shape.js_
```javascript
class Shape {
constructor() {
this.color = 'red';
}
colorLog() {
console.log(this.color);
}
}
export default Shape;
```
_Circle.js_
```javascript
import Shape from "./Shape";
class Circle extends Shape {
constructor() {
super();
// FIXME: description should show as unused in VSCode, as it does in WebStorm.
this.description = 'A circle shape';
}
// FIXME: circleLog should show as unused in VSCode, as it does in WebStorm.
circleLog() {
// NOTE: both VSCode and WebStorm have detected an unused variable.
const num = 2;
// NOTE: colorLog is being used, so no unused code errors in Shape.js file.
super.colorLog();
}
}
// FIXME: export should show as unused in VSCode, as it does in WebStorm.
export default Circle;
```
**VSCode Screen**
<img width="1440" alt="screen shot 2019-01-04 at 9 23 48 pm" src="https://user-images.githubusercontent.com/12591890/50719791-161a2980-1067-11e9-8b16-3f4e4fe6b531.png">
**WebStorm Screen**
<img width="1440" alt="screen shot 2019-01-04 at 9 23 07 pm" src="https://user-images.githubusercontent.com/12591890/50719793-1ca8a100-1067-11e9-8c17-071746dd085f.png">
| Suggestion,In Discussion | high | Critical |
396,628,799 | TypeScript | Rename file when renaming default export | Currently we can use the `rename symbol` command and that will rename the symbol and its usages (exports, imports, usages in other files, etc.). That's a great start, but I'd love to see this feature expanded to do the following:
- Many times a file name will coincide with the symbol being refactored. The refactor option should be available when renaming the file, so everything can be done at once.
- In addition to the refactor changing symbol references across all files, it'd be nice to have the ability to also update comments, strings, etc. that refer to that symbol.
**Code Example:**
_Shape.js_
```javascript
class Shape {}
export default Shape;
```
_Circle.js - We reference Shape as an import, extends, inside a comment, and inside a string. They should all be refactored._
```javascript
import Shape from "./Shape";
// Shape subclass
class Circle extends Shape {
constructor() {
super();
console.log('A circle is a Shape.');
}
}
export default Circle;
```
**WebStorm Examples**
<img width="1440" alt="screen shot 2019-01-04 at 8 36 22 pm" src="https://user-images.githubusercontent.com/12591890/50719461-c1c07b00-1061-11e9-96f2-768c81458e24.png">
<img width="1440" alt="screen shot 2019-01-04 at 8 37 02 pm" src="https://user-images.githubusercontent.com/12591890/50719462-c1c07b00-1061-11e9-9f82-1a2af9ca3ba7.png">
| Suggestion,Needs Proposal,Domain: Refactorings | low | Minor |
396,629,303 | go | x/build/cmd/gopherbot: when closing backport issues, ignores "Fixes" vs "For"/"Updates" verb before issue mention | Gopherbot closes backport issues once it detects that an associated commit has been merged. This works well if the backport only requires one commit, but causes issues when there are multiple. Gopherbot should only close the issue if the "Fixes #" label exists in the commit message.
See #29565 for an example of Gopherbot in action.
One possible solution would be to add a new field to the [GerritCL](https://godoc.org/golang.org/x/build/maintner#GerritCL) type that indicates whether or not this CL "fixes" the issue, or just updates/relates to it.
@golang/osp-team | Builders,NeedsFix | low | Minor |
396,665,884 | TypeScript | Option to disable decorator transform | ## Search Terms
decorator output
<!-- List of keywords you searched for before creating this issue. Write them down here so that others can find this suggestion more easily -->
## Suggestion
Decorators are always transformed. In some cases we critically need to preserve decorators in the output to feed into other tools, such as Babel.
All the other issues around this are closed, so I can't see what the plan is here. Adding a new flag to disable decorator transformation should be forward and backward compatible with any other implementation of decorators, old or new proposal.
## Use Cases
- Use Babel 7 decorator support to compile the current decorator proposal
- Feed tsc output into other analysis tools that expect decorators in JS source.
## Examples
<!-- Show how this would be used and what the behavior would be -->
## Checklist
My suggestion meets these guidelines:
* [x] This wouldn't be a breaking change in existing TypeScript/JavaScript code
* [x] This wouldn't change the runtime behavior of existing JavaScript code
* [x] This could be implemented without emitting different JS based on the types of the expressions
* [x] This isn't a runtime feature (e.g. library functionality, non-ECMAScript syntax with JavaScript output, etc.)
* [x] This feature would agree with the rest of [TypeScript's Design Goals](https://github.com/Microsoft/TypeScript/wiki/TypeScript-Design-Goals).
| Suggestion,Awaiting More Feedback | low | Major |
396,672,755 | rust | `&mut &T` coerced to `&T` suggests `&mut mut x` | This code ([play](https://play.rust-lang.org/?version=nightly&mode=debug&edition=2018&gist=6c08c4287f618e5377a8443c41982863)):
```rust
struct X;
impl X {
fn mutate(&mut self) {}
}
fn main() {
let term = Some(X);
let term = match &term {
Some(term) => &mut term,
None => term.as_ref().unwrap(),
};
term.mutate();
}
```
Produces these errors:
```
error[E0596]: cannot borrow `term` as mutable, as it is not declared as mutable
--> src/main.rs:9:23
|
9 | Some(term) => &mut term,
| ^^^^^^^^^
| |
| cannot borrow as mutable
| try removing `&mut` here
error[E0596]: cannot borrow `*term` as mutable, as it is behind a `&` reference
--> src/main.rs:12:5
|
9 | Some(term) => &mut term,
| --------- help: consider changing this to be a mutable reference: `&mut mut term`
...
12 | term.mutate();
| ^^^^ `term` is a `&` reference, so the data it refers to cannot be borrowed as mutable
error: aborting due to 2 previous errors
```
Note that the second error suggests changing `&mut term` to `&mut mut term`, which obviously won't fix the issue. | T-compiler,A-NLL,A-suggestion-diagnostics,D-confusing | medium | Critical |
396,693,536 | neovim | sockconnect: add support for connecting to FIFO | <!-- Before reporting: search existing issues and check the FAQ. -->
- `nvim --version`: 0.3.2
- Vim (version: ) behaves differently? N/A
- Operating system/version: macOS 10.13.6
- Terminal name/version: Kitty 0.13.2
- `$TERM`: screen-256color
### Steps to reproduce using `nvim -u NORC`
1) `mkfifo /tmp/myfifo`
2) Open `nvim -u NORC test.vim` and save the buffer
3) Copy the following script and the source the buffer
```vim
function! s:OnData(id, data, event)
echo join(a:data, '\n')
endfunction
let id = sockconnect('pipe', '/tmp/myfifo', { 'on_data': function('s:OnData') })
```
The pipe works as expected when running the following from a shell.
```shell
echo "hello" > /tmp/myfifo &
cat /tmp/myfifo
```
### Actual behaviour
Neovim throws this error: `connection failed: connection refused`
### Expected behaviour
Be able to connect to the named pipe.
| enhancement,channels-rpc | low | Critical |
396,700,674 | pytorch | Possible regression in incremental build | ## ๐ Bug
Running `python setup.py rebuild develop` constantly re-installs PyTorch. This regresses the time of our no-op build. I just noticed this today. It might be a regression.
## To Reproduce
Directly run ninja install in the build directory. Observe it always thinks something is dirty:
```
(base) [[email protected] /data/users/zdevito/pytorch] cd build
(base) [[email protected] /data/users/zdevito/pytorch/build] ninja install
[0/1] Install the project...
-- Install configuration: "RelWithDebInfo"
(base) [[email protected] /data/users/zdevito/pytorch/build] ninja install
[0/1] Install the project...
-- Install configuration: "RelWithDebInfo"
(base) [[email protected] /data/users/zdevito/pytorch/build] ninja -d explain install
ninja explain: output CMakeFiles/install.util doesn't exist
ninja explain: CMakeFiles/install.util is dirty
[0/1] Install the project...
-- Install configuration: "RelWithDebInfo"
```
cc @malfet @seemethere @walterddr | module: build,triaged | low | Critical |
396,762,989 | scrcpy | Any ability to send, e.g., ctrl+c as key entry? | First, this is awesome; thanks for your contribution. I use Termux (terminal emulator and linux app). Is there a way to send control key combinations as keystrokes? E.g., so I can ctrl+C to kill a program from the command line in Termux?
Thanks again. | feature request | low | Minor |
396,786,559 | react | Add `get` function to `useState` | <!--
Note: if the issue is about documentation or the website, please file it at:
https://github.com/reactjs/reactjs.org/issues/new
-->
**Do you want to request a *feature* or report a *bug*?**
- feature
**What is the current behavior?**
Code from [Introducing Hooks](https://reactjs.org/docs/hooks-intro.html):
```javascript
import { useState } from 'react';
function Example() {
// Declare a new state variable, which we'll call "count"
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
```
```javascript
// each time "count" changed, this arrow function will be created again.
// so that it can access the latest "count"
onClick={() => setCount(count + 1)}
```
I don't think it is good to create a fixed function many times, so I try to modify the code:
(Update on Jul 2022: No matter using the inline anonymous function or wrapping with `useCallback`, the function will always be created. The difference is that, in `useCallback` approach, the function reference will not be changed, which could be helpful if we use `memo` to wrap the component who receives the function as a property)
```javascript
const [count, setCount] = useState(0);
const handleClick = useCallback(() => setCount(count + 1), []);
```
But obviously the callback in `useCallback` couldn't get the latest `count` because I pass in an empty inputs array to avoid this callback been generated again and again.
So, in fact, the inputs array decide two things:
1. when to recreate the callback
2. which state can be accessed in the callback
In most situation, the two things are one thing, but here they conflict.
So I think maybe it's good to add a `get` function to `useState` like this:
```javascript
import { useState, useCallback } from 'react';
function Example() {
// Declare a new state variable, which we'll call "count"
const [count, setCount, getCount] = useState(0);
const handleClick = useCallback(() => setCount(getCount() + 1), []);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={handleClick}>
Click me
</button>
</div>
);
}
```
Maybe it's confusing because `getCount` can totally replace `count`, but it brings the possible to avoid creating callbacks again and again.
### Edited
https://github.com/facebook/react/issues/14543#issuecomment-452237355 exactly resolves the case above. But thereโre many other scenarios can't use `updater` to resolve. Here are some more code snippets:
#### 1. Access states in a timer.
```javascript
useEffect(() => {
// or setInterval
const id = setTimeout(() => {
// access states
}, period);
return () => clearTimeout(id);
}, inputs);
```
#### 2. Access states in WebSocket callbacks
```javascript
useEffect(() => {
// create a WebSocket client named "ws"
ws.onopen = () => {
// access states
};
ws.onmessage = () => {
// access states
};
return () => ws.close();
}, inputs);
```
#### 3. Access states in Promise
```javascript
useEffect(() => {
create_a_promise().then(() => {
// access states
});
}, inputs);
```
#### 4. Access states in event callbacks
```javascript
useEffect(() => {
function handleThatEvent() {
// access states
}
instance.addEventListener('eventName', handleThatEvent);
return instance.removeEventListener('eventName', handleThatEvent);
}, inputs);
```
We had to use some workaround patterns to resolve those cases, like
https://github.com/facebook/react/issues/14543#issuecomment-452676760
https://github.com/facebook/react/issues/14543#issuecomment-453058025
https://github.com/facebook/react/issues/14543#issuecomment-453079958
Or a funny way:
```javascript
const [state, setState] = useState();
useEffect(() => {
// or setInterval
const id = setTimeout(() => {
// access states
setState((prevState) => {
// Now I can do anything with state...๐คฎ
...
return prevState;
});
}, period);
return () => clearTimeout(id);
}, inputs);
```
So let's discuss and wait...
https://github.com/facebook/react/issues/14543#issuecomment-452713416
**If the current behavior is a bug, please provide the steps to reproduce and if possible a minimal demo of the problem. Your bug will get fixed much faster if we can run your code and it doesn't have dependencies other than React. Paste the link to your JSFiddle (https://jsfiddle.net/Luktwrdm/) or CodeSandbox (https://codesandbox.io/s/new) example below:**
**What is the expected behavior?**
**Which versions of React, and which browser / OS are affected by this issue? Did this work in previous versions of React?**
- React 16.7.0-alpha.2
| Type: Discussion | medium | Critical |
396,792,794 | pytorch | No test coverage for kwargs of AvgPool2d and AvgPool3d | `ceil_mode` and `count_include_pad` are not tested at all.
cc @albanD @mruberry | module: bootcamp,module: nn,triaged,module: pooling | low | Minor |
396,797,135 | pytorch | AvgPool2d doesn't test if kernel is smaller than input size | AvgPool3d is OK
```
>>> import torch
>>> m = torch.nn.AvgPool2d(3)
>>> x = torch.zeros(1,2,2)
>>> m(x)
tensor([[[0.]]])
>>> m = torch.nn.AvgPool3d(3)
>>> x = torch.zeros(1,2,2,2)
>>> m(x)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "/Users/ezyang/Dev/pytorch-tmp/torch/nn/modules/module.py", line 489, in __call__
result = self.forward(*input, **kwargs)
File "/Users/ezyang/Dev/pytorch-tmp/torch/nn/modules/pooling.py", line 638, in forward
self.padding, self.ceil_mode, self.count_include_pad)
RuntimeError: invalid argument 2: input image (T: 2 H: 2 W: 2) smaller than kernel size (kT: 3 kH: 3 kW: 3) at ../aten/src/THNN/generic/VolumetricAveragePooling.c:58
``` | module: bootcamp,module: error checking,triaged,better-engineering,module: pooling | low | Critical |
396,830,228 | rust | Properly test cross-language LTO | I'd like us to stabilize cross-language LTO as soon as possible but before we can do that we need more robust tests. Some background:
- Cross-language LTO works by emitting LLVM bitcode instead of machine code for RLIBs/staticlibs and then lets the linker do the final ThinLTO pass. Because code coming from other languages (most notably C/C++) are also compiled to LLVM bitcode, the linker can perform inter-procedural optimizations across language boundaries.
- For this to work properly, the LLVM versions used by `rustc` and the foreign language compilers must roughly be the same (at least the same major version).
- The current implementation of cross-language LTO is available via `-Zcross-lang-lto` in `rustc`. It has some tests that make sure libraries indeed contain LLVM bitcode instead of object files. This is the bare-minimum requirement for cross-language LTO to work.
However, there is a problem with this approach:
- Having LLVM bitcode available might not be sufficient for cross-language optimizations to actually happen. For example, LLVM will not inline one function into another if their target-features don't match. For this reason we unconditionally emit the `target-cpu` attribute for LLVM functions. For now this seems to be sufficient but for future versions of LLVM additional requirements might emerge and break cross-language LTO in a silent way (i.e. everything still compiles but the expected optimizations aren't happening).
So, to test the feature in a more robust way, we have to have a test that actually compiles some mixed Rust/C++ code and then verifies that function inlining across language boundaries was successful.
The good news is that trivial functions (e.g. ones that just return a constant integer) are reliably inlined, so we have a good way of checking whether inlining happens at all.
The bad news is that, since Rust's LLVM is ahead of stable Clang releases, we need to build our own Clang in order to get a compatible version. We already have Clang in tree because we need it for building Rust-enabled LLDB, but this is not the default.
So my current proposal for implementing these tests is:
- Add a script (available to `run-make-fulldeps` tests) that checks if a compatible Clang is available.
- Add an option to `compiletest` that allows to specify whether tests relying on Clang are optional or required.
- If Clang-based tests are required, fail them if Clang is not available, otherwise just ignore them (so that local testing can be done without also building Clang).
- Force building Rust LLDB on major platform CI jobs, thus making Clang available.
- For those same jobs, set the `compiletest` option that requires Clang to be available.
This way cross-language LTO will be tested by CI and hopefully `sccache` will make building Clang+LLDB cheap enough. But we also rely on not forgetting to set the appropriate flags in CI images, which might cause things to silently go untested.
@rust-lang/infra & @rust-lang/compiler, do you have any thoughts? Or a better, less complicated approach? It would be great if we could require Clang unconditionally but I'm afraid that that would be too much of a burden for people running tests locally. | A-linkage,A-testsuite,T-compiler,E-help-wanted,T-bootstrap,T-infra | low | Major |
396,983,697 | pytorch | computing entropy of a tensor | I think it is very useful if we can have feature enhancement that we can compute the entropy of a tensor, the similar way that we can do it for mean, std, etc
in more details:
It would be super useful to have a function that compute the entropy of a tensor. well, saying that, it is good if that function can compute the entropy in different ways depending on our desire; meaning that we can define a dimension and compute the entropy based on that, e.g. computiong the entropy of a tensor channel wise, or etc
This request was first made [Here](https://github.com/pytorch/vision/issues/662#issuecomment-442036469) and then [here](https://github.com/pytorch/contrib/issues/16), but I am told that it will be more useful to open it here
cc @ezyang @gchanan @zou3519 @bdhirsh @jbschlosser | high priority,triaged,function request | medium | Major |
397,026,721 | go | gccgo: incorrect call stack from runtime.CallersFrames | Stack traces are not quite right for gccgo with optimizations on (mid-stack inlining, particularly).
```
package main
import (
"fmt"
"runtime"
)
func main() {
f()
}
func f() {
g()
}
//go:noinline
func g() {
for i := 0; i < 4; i++ {
var pcs [1]uintptr
runtime.Callers(i+1, pcs[:])
f, _ := runtime.CallersFrames(pcs[:]).Next()
fmt.Printf("%x %s %s:%d\n", f.PC, f.Function, f.File, f.Line)
}
}
```
Running under gc, I get:
```
48683c main.g /usr/local/google/home/khr/gowork/tmp5.go:19
48678f main.f /usr/local/google/home/khr/gowork/tmp5.go:12
48675f main.main /usr/local/google/home/khr/gowork/tmp5.go:9
428846 runtime.main /usr/local/google/home/khr/go1.11/src/runtime/proc.go:201
```
Running under gccgo -O3, I get:
```
56261c5ebc19 main.g /usr/local/google/home/khr/gowork/tmp5.go:19
56261c5ebeb3 main.f /usr/local/google/home/khr/gowork/tmp5.go:12
56261c5ebeb3 main.f /usr/local/google/home/khr/gowork/tmp5.go:12
7f9974ede51c runtime_main ../../../src/libgo/runtime/proc.c:606
```
Note the third line - that should be `main.main`, not a second copy of `main.f`.
gccgo works without -O3.
Mid-stack inlining is happening with -O3, `main.main` calls `main.g` directly. `main.f` isn't even in the binary.
It doesn't help to provide `runtime.Callers` a larger buffer.
Note that this code does work, calling `runtime.Callers` just once and using the `runtime.CallersFrames` iterator:
```
package main
import (
"fmt"
"runtime"
)
func main() {
f()
}
func f() {
g()
}
//go:noinline
func g() {
var pcs [10]uintptr
n := runtime.Callers(1, pcs[:])
iter := runtime.CallersFrames(pcs[:n])
for {
f, more := iter.Next()
fmt.Printf("%x %s %s:%d\n", f.PC, f.Function, f.File, f.Line)
if !more {
break
}
}
}
```
```
55bb3972acd4 main.g /usr/local/google/home/khr/gowork/tmp6.go:18
55bb3972afc3 main.f /usr/local/google/home/khr/gowork/tmp6.go:12
55bb3972afc3 main.main /usr/local/google/home/khr/gowork/tmp6.go:9
7fa2706d451c runtime_main ../../../src/libgo/runtime/proc.c:606
```
@ianlancetaylor
| NeedsInvestigation | low | Minor |
397,119,201 | vscode | Allow more powerful onEnterRules for cursor alignment | In the Python extension, we'd like to add support for variable-length indents on enter to better support PEP8 formatting (Microsoft/vscode-python#481 and others). For example:
```python
def func(a,
|
```
Hitting enter on the first line should stick the cursor where `|` is, not just a single indent. In this second case, it should do a single indent:
```python
def func(
|
```
There appears to only be two ways to handle this:
- `onEnterRules`
- On-type formatting
We'd like to avoid the latter, as we currently support both Jedi and our own language server, which means implementing on-type formatting in two different places (since a single language cannot have multiple on-type formatting providers). Plus, on-type formatting is not enabled by default nor is very popular (and enables a slew of formatting rules other than cursor alignment), so forcing users to enable on-type formatting as a whole would be good to avoid.
The other candidate is `onEnterRules`, but it's not powerful enough to be able to do something like this, as:
- The "actions" work in terms of indents, whereas these cursor positions may not be a round multiple of an indent, meaning `appendText` would be required, but...
- `appendText` is only allowed to be a static string, and cannot be generated on the fly.
- Rules are applied using regex to deduce context, which gets unweildy, and even more difficult without #50952.
- Even with something like #17281, there wouldn't be a way to convert a capture group to "whitespace of the same length of the capture group" (plus the issue of tab indents).
My initial thought was to allow a function to be provided in `onEnterRules` instead of just specifying `appendText`. For example, something like:
```ts
{
beforeText: /\s+def\s+\w+\(\w+/, /* not the real regex */
action: {
indentAction: IndentAction.None,
appendText: (document, position, formatOptions, ... /* something */) => calculateIndent(...) // returns a string
}
}
```
However, on closer inspection of how `onEnterRules` works, it appears that it needs to be serializable, so I'm not sure it'd be able to have a callback like the above.
Is there something that can be added to allow more powerful rules on-enter? Or, is there something that already exists that we've missed? | feature-request,editor-input,editor-autoindent | medium | Critical |
397,148,426 | godot | Issues with new Polygon2D UV Editor features | <!-- Please search existing issues for potential duplicates before filing yours:
https://github.com/godotengine/godot/issues?q=is%3Aissue
-->
**Godot version:**
e46f28e
**Issue description:**
@reduz
- The new internal vertex feature seems unintuitive, at least to me.
- When a internal vertex is present, the polygon editor in the 2D editor will treat the polygon as having no points.
- <s>You can't undo internal vertex creation, or custom polygon removal via UndoRedo.</s> Fixed by #24899.
- <s>The lines of the actual polygon are hidden when any custom polygon is present.</s> Fixed by 7f69da488e971b035fbdf044536db1ce9a100d50.
- Polygon creation with <s>either internal vertexes or</s> custom polygons present is buggy. Partially fixed by 96445cd. | bug,enhancement,topic:editor,confirmed | medium | Critical |
397,151,463 | pytorch | DataLoader with option to re-use worker processes | ## ๐ Feature
Currently after an epoch is ended dataloader spawns a new process to read data. This means if processes have cached some internal state (db connection, indexing, ...) they will be lost and the new process will have the overhead of creating the connection or indexing.
## Motivation
I have a custom dataset (that indexes data for fast retrieval) and only part of the dataset is used in training. If I have a training with longer epochs, training will be faster than shorter epochs because when an epoch ends a new process will have to re-read and index the custom dataset.
## Pitch
A flag to tell dataloader to re-use the worker processes. If the process pool has the re-use option ([Loky from joblib](https://github.com/tomMoral/loky) does) that would be enough.
## Alternatives
Documentation should specify this issue. Option to use [Loky](https://github.com/tomMoral/loky) for all multi-processing backend will be also great.
## Additional context
Started a discussion [here](https://discuss.pytorch.org/t/dataloader-re-use-worker-processes/34071)
cc @ezyang @gchanan @SsnL | high priority,feature,module: dataloader,triaged | high | Critical |
397,176,880 | angular | Animation: Automatic property calculation ('!') not working when using AnimationPlayer/AnimationBuilder | <!--๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
Oh hi there! ๐
To expedite issue processing please search open and closed issues before submitting a new one.
Existing issues often contain information about workarounds, resolution, or progress updates.
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
๐
-->
# ๐ bug report
### Affected Package
<!-- Can you pin-point one or more @angular/* packages as the source of the bug? -->
<!-- โ๏ธedit: --> The issue is caused by package: @angular/animations
### Is this a regression?
<!-- Did this behavior use to work in the previous version? -->
<!-- โ๏ธ--> I'm not sure
### Description
Given the following animation, for expanding an element:
```
animate(300, style({
height: '!'
}))
```
it fails when consuming it with AnimationBuilder and trying to run it using AnimationPlayer (while it works perfectly fine using triggers with state & transition).
## ๐ฌ Minimal Reproduction
https://stackblitz.com/edit/angular-issue-repro2-3gdmsb?file=src%2Fapp%2Fapp.component.html
<!--
If StackBlitz is not suitable for reproduction of your issue, please create a minimal GitHub repository with the reproduction of the issue. Share the link to the repo below along with step-by-step instructions to reproduce the problem, as well as expected and actual behavior.
Issues that don't have enough info and can't be reproduced will be closed.
You can read more about issue submission guidelines here: https://github.com/angular/angular/blob/master/CONTRIBUTING.md#-submitting-an-issue
-->
## ๐ฅ Exception or Error
<pre><code>
Invalid keyframe value for property height: undefined.
ERROR DOMException: Failed to execute 'animate' on 'Element': Partial keyframes are not supported.
at WebAnimationsPlayer._triggerWebAnimation
</code></pre>
## ๐ Your Environment
**Angular Version:**
<pre><code>
Angular CLI: 7.2.0
Node: 10.15.0
OS: win32 x64
Angular: 7.2.0
... animations, common, compiler, compiler-cli, core, forms
... language-service, platform-browser, platform-browser-dynamic
... router
Package Version
-----------------------------------------------------------
@angular-devkit/architect 0.12.0
@angular-devkit/build-angular 0.12.0
@angular-devkit/build-optimizer 0.12.0
@angular-devkit/build-webpack 0.12.0
@angular-devkit/core 7.2.0
@angular-devkit/schematics 7.2.0
@angular/cli 7.2.0
@ngtools/webpack 7.2.0
@schematics/angular 7.2.0
@schematics/update 0.12.0
rxjs 6.3.3
typescript 3.1.6
</code></pre>
| type: bug/fix,area: animations,freq3: high,P3 | low | Critical |
397,203,229 | go | runtime: unexpected signal on Termux android/arm64 | <!-- Please answer these questions before submitting your issue. Thanks! -->
### What version of Go are you using (`go version`)?
<pre>
$ go version
go version go1.11.4 android/arm64
</pre>
### Does this issue reproduce with the latest release?
Yes
### What operating system and processor architecture are you using (`go env`)?
<details><summary><code>go env</code> Output</summary><br><pre>
$ go env
GOARCH="arm64"
GOBIN=""
GOCACHE="/data/data/com.termux/files/home/.cache/go-build"
GOEXE=""
GOFLAGS=""
GOHOSTARCH="arm64"
GOHOSTOS="android"
GOOS="android"
GOPATH="/data/data/com.termux/files/home/go"
GOPROXY=""
GORACE=""
GOROOT="/data/data/com.termux/files/usr/lib/go"
GOTMPDIR=""
GOTOOLDIR="/data/data/com.termux/files/usr/lib/go/pkg/tool/android_arm64"
GCCGO="gccgo"
CC="aarch64-linux-android-clang"
CXX="aarch64-linux-android-clang++"
CGO_ENABLED="1"
GOMOD="/data/data/com.termux/files/home/storage/shared/dev/projects/playground/go.mod"
CGO_CFLAGS="-g -O2"
CGO_CPPFLAGS=""
CGO_CXXFLAGS="-g -O2"
CGO_FFLAGS="-g -O2"
CGO_LDFLAGS="-g -O2"
PKG_CONFIG="/home/builder/.termux-build/_cache/18-aarch64-21-v1/bin/aarch64-linux-android-pkg-config"
GOGCCFLAGS="-fPIC -pthread -fno-caret-diagnostics -Qunused-arguments -fmessage-length=0 -fdebug-prefix-map=/data/data/com.termux/files/usr/tmp/go-build261125908=/tmp/go-build -gno-record-gcc-switches"
</pre></details>
### What did you do?
<!--
If possible, provide a recipe for reproducing the error.
A complete runnable program is good.
A link on play.golang.org is best.
-->
I create project outside the gopath using go mod
```
go mod init github.com/uudashr/playground
```
and have this files `doc.go` and `hello_test.go`
```go
package playground_test
import "testing"
func TestHello(t *testing.T) {
// Do nothing
}
```
then run test
```
go test
```
### What did you expect to see?
Always shows
```
PASS
ok github.com/uudashr/playground 0.038s
```
### What did you see instead?
1 out of 10 stuck/hung
and
1 out of 10 shows
```
PASS
ok github.com/uudashr/playground 0.033s
fatal error: unexpected signal during runtime execution
[signal SIGSEGV: segmentation violation code=0x1 addr=0x64 pc=0x7e1195d20]
runtime stack:
runtime.throw(0x7e154c90f, 0x2a)
/data/data/com.termux/files/usr/lib/go/src/runtime/panic.go:608 +0x4c
runtime.sigpanic()
/data/data/com.termux/files/usr/lib/go/src/runtime/signal_unix.go:374 +0x2a4
runtime.markrootSpans(0x4000031270, 0x0)
/data/data/com.termux/files/usr/lib/go/src/runtime/mgcmark.go:357 +0x88
runtime.markroot(0x4000031270, 0x200000004)
/data/data/com.termux/files/usr/lib/go/src/runtime/mgcmark.go:225 +0x340
runtime.gcDrain(0x4000031270, 0xd)
/data/data/com.termux/files/usr/lib/go/src/runtime/mgcmark.go:882 +0x108
runtime.gcBgMarkWorker.func2()
/data/data/com.termux/files/usr/lib/go/src/runtime/mgc.go:1862 +0x16c
runtime.systemstack(0x73ca02f400)
/data/data/com.termux/files/usr/lib/go/src/runtime/asm_arm64.s:230 +0x90
runtime.mstart()
/data/data/com.termux/files/usr/lib/go/src/runtime/proc.go:1229
goroutine 50 [GC worker (idle)]:
runtime.systemstack_switch()
/data/data/com.termux/files/usr/lib/go/src/runtime/asm_arm64.s:178 +0x8 fp=0x40002d6f50 sp=0x40002d6f40 pc=0x7e11ca290
runtime.gcBgMarkWorker(0x4000030000)
/data/data/com.termux/files/usr/lib/go/src/runtime/mgc.go:1826 +0x198 fp=0x40002d6fd0 sp=0x40002d6f50 pc=0x7e1193ae0
runtime.goexit()
/data/data/com.termux/files/usr/lib/go/src/runtime/asm_arm64.s:1114 +0x4 fp=0x40002d6fd0 sp=0x40002d6fd0 pc=0x7e11cca0c
created by runtime.gcBgMarkStartWorkers
/data/data/com.termux/files/usr/lib/go/src/runtime/mgc.go:1720 +0x70
goroutine 1 [semacquire]:
sync.runtime_Semacquire(0x4000253a38)
/data/data/com.termux/files/usr/lib/go/src/runtime/sema.go:56 +0x2c
sync.(*WaitGroup).Wait(0x4000253a30)
/data/data/com.termux/files/usr/lib/go/src/sync/waitgroup.go:130 +0x64
cmd/go/internal/work.(*Builder).Do(0x40003e6280, 0x40003d3540)
/data/data/com.termux/files/usr/lib/go/src/cmd/go/internal/work/exec.go:174 +0x31c
cmd/go/internal/test.runTest(0x7e1aef420, 0x400000e070, 0x0, 0x0)
/data/data/com.termux/files/usr/lib/go/src/cmd/go/internal/test/test.go:759 +0xcd8
main.main()
/data/data/com.termux/files/usr/lib/go/src/cmd/go/main.go:219 +0x6e8
goroutine 5 [syscall]:
os/signal.signal_recv(0x4000000000)
/data/data/com.termux/files/usr/lib/go/src/runtime/sigqueue.go:139 +0xb8
os/signal.loop()
/data/data/com.termux/files/usr/lib/go/src/os/signal/signal_unix.go:23 +0x18
created by os/signal.init.0
/data/data/com.termux/files/usr/lib/go/src/os/signal/signal_unix.go:29 +0x30
goroutine 68 [select]:
cmd/go/internal/work.(*Builder).Do.func2(0x4000253a30, 0x40003e6280, 0x400059b2c0)
/data/data/com.termux/files/usr/lib/go/src/cmd/go/internal/work/exec.go:155 +0xdc
created by cmd/go/internal/work.(*Builder).Do
/data/data/com.termux/files/usr/lib/go/src/cmd/go/internal/work/exec.go:152 +0x2f8
goroutine 69 [select]:
cmd/go/internal/work.(*Builder).Do.func2(0x4000253a30, 0x40003e6280, 0x400059b2c0)
/data/data/com.termux/files/usr/lib/go/src/cmd/go/internal/work/exec.go:155 +0xdc
created by cmd/go/internal/work.(*Builder).Do
/data/data/com.termux/files/usr/lib/go/src/cmd/go/internal/work/exec.go:152 +0x2f8
goroutine 60 [chan receive]:
cmd/go/internal/base.processSignals.func1(0x400002c960)
/data/data/com.termux/files/usr/lib/go/src/cmd/go/internal/base/signal.go:21 +0x24
created by cmd/go/internal/base.processSignals
/data/data/com.termux/files/usr/lib/go/src/cmd/go/internal/base/signal.go:20 +0x78
goroutine 70 [select]:
cmd/go/internal/work.(*Builder).Do.func2(0x4000253a30, 0x40003e6280, 0x400059b2c0)
/data/data/com.termux/files/usr/lib/go/src/cmd/go/internal/work/exec.go:155 +0xdc
created by cmd/go/internal/work.(*Builder).Do
/data/data/com.termux/files/usr/lib/go/src/cmd/go/internal/work/exec.go:152 +0x2f8
goroutine 71 [select]:
cmd/go/internal/work.(*Builder).Do.func2(0x4000253a30, 0x40003e6280, 0x400059b2c0)
/data/data/com.termux/files/usr/lib/go/src/cmd/go/internal/work/exec.go:155 +0xdc
created by cmd/go/internal/work.(*Builder).Do
/data/data/com.termux/files/usr/lib/go/src/cmd/go/internal/work/exec.go:152 +0x2f8
goroutine 72 [runnable]:
syscall.Syscall(0x23, 0xffffffffffffff9c, 0x400043a280, 0x0, 0x0, 0x400043a280, 0x0)
/data/data/com.termux/files/usr/lib/go/src/syscall/asm_linux_arm64.s:9 +0x8
syscall.unlinkat(0xffffffffffffff9c, 0x400043a1e0, 0x4a, 0x0, 0x0, 0x400043a1e0)
/data/data/com.termux/files/usr/lib/go/src/syscall/zsyscall_linux_arm64.go:126 +0x68
syscall.Unlink(0x400043a1e0, 0x4a, 0x4a, 0x400043a1e0)
/data/data/com.termux/files/usr/lib/go/src/syscall/syscall_linux.go:168 +0x34
os.Remove(0x400043a1e0, 0x4a, 0xe, 0x4a)
/data/data/com.termux/files/usr/lib/go/src/os/file_unix.go:306 +0x28
os.RemoveAll(0x400043a1e0, 0x4a, 0x3b, 0x7e1535c2e)
/data/data/com.termux/files/usr/lib/go/src/os/path.go:68 +0x28
os.RemoveAll(0x400033b4c0, 0x3b, 0x40000e8ca8, 0x7e1472198)
/data/data/com.termux/files/usr/lib/go/src/os/path.go:109 +0x390
cmd/go/internal/test.builderCleanTest(0x40003e6280, 0x40000c4780, 0x40003e62f0, 0x7e1762840)
/data/data/com.termux/files/usr/lib/go/src/cmd/go/internal/test/test.go:1603 +0x4c
cmd/go/internal/work.(*Builder).Do.func1(0x40000c4780)
/data/data/com.termux/files/usr/lib/go/src/cmd/go/internal/work/exec.go:107 +0x50
cmd/go/internal/work.(*Builder).Do.func2(0x4000253a30, 0x40003e6280, 0x400059b2c0)
/data/data/com.termux/files/usr/lib/go/src/cmd/go/internal/work/exec.go:165 +0x8c
created by cmd/go/internal/work.(*Builder).Do
/data/data/com.termux/files/usr/lib/go/src/cmd/go/internal/work/exec.go:152 +0x2f8
goroutine 73 [select]:
cmd/go/internal/work.(*Builder).Do.func2(0x4000253a30, 0x40003e6280, 0x400059b2c0)
/data/data/com.termux/files/usr/lib/go/src/cmd/go/internal/work/exec.go:155 +0xdc
created by cmd/go/internal/work.(*Builder).Do
/data/data/com.termux/files/usr/lib/go/src/cmd/go/internal/work/exec.go:152 +0x2f8
``` | NeedsInvestigation,compiler/runtime | medium | Critical |
397,238,376 | pytorch | Deformable Convolution | ## ๐ Feature
Exactly as introduced in Microsoft paper : https://arxiv.org/pdf/1703.06211.pdf
## Motivation
This operator has great advantages and abilities, and his popularity is increasing.
I don't think that any further explanation is needed.
BTW, other frameworks already have this operator, and I'm not talking about private people githubs.. | feature,triaged,module: vision | low | Major |
397,276,521 | pytorch | RuntimeError: [enforce fail at pybind_state.cc:1111] success. Error running net train | Learn caffe2 to train MNIST-dataset. This network error occurs. Why?
[E prefetch_op.h:110] Prefetching error Unknown data type.
[E prefetch_op.h:83] Prefetching failed.
[E net_simple.cc:68] Operator failed: input: "dbreader_/home/tcl/caffe2_notebooks/tutorial_data/mnist/mnist-train-nchw-lmdb" output: "data_uint8" output: "label" name: "" type: "TensorProtosDBInput" arg { name: "batch_size" i: 64 } device_option { device_type: 0 device_id: 0 }
WARNING:caffe2.python.workspace:Original python traceback for operator `-1818544912` in network `train` in exception above (most recent call last):
Traceback (most recent call last):
File "/home/tcl/PycharmProjects/caffe2/mnist_caffe2.py", line 285, in <module>
workspace.RunNet(train_model.net.Proto().name)
File "/usr/local/lib/python2.7/dist-packages/caffe2/python/workspace.py", line 236, in RunNet
StringifyNetName(name), num_iter, allow_fail,
File "/usr/local/lib/python2.7/dist-packages/caffe2/python/workspace.py", line 197, in CallWithExceptionIntercept
return func(*args, **kwargs)
RuntimeError: [enforce fail at pybind_state.cc:1111] success. Error running net train
frame #0: c10::ThrowEnforceNotMet(char const*, int, char const*, std::__cxx11::basic_string<char, std::char_traits<char>, std::allocator<char> > const&, void const*) + 0x78 (0x7f1208875408 in /usr/local/lib/python2.7/dist-packages/caffe2/python/../../torch/lib/libc10.so)
frame #1: <unknown function> + 0x424df (0x7f120baa54df in /usr/local/lib/python2.7/dist-packages/caffe2/python/caffe2_pybind11_state.so)
frame #2: <unknown function> + 0x86831 (0x7f120bae9831 in /usr/local/lib/python2.7/dist-packages/caffe2/python/caffe2_pybind11_state.so)
frame #3: PyEval_EvalFrameEx + 0x695b (0x558f90aa78db in /usr/bin/python2.7)
frame #4: PyEval_EvalCodeEx + 0x6da (0x558f90a9ed0a in /usr/bin/python2.7)
frame #5: PyEval_EvalFrameEx + 0x5cb8 (0x558f90aa6c38 in /usr/bin/python2.7)
frame #6: PyEval_EvalCodeEx + 0x6da (0x558f90a9ed0a in /usr/bin/python2.7)
frame #7: PyEval_EvalFrameEx + 0x567e (0x558f90aa65fe in /usr/bin/python2.7)
frame #8: PyEval_EvalCodeEx + 0x6da (0x558f90a9ed0a in /usr/bin/python2.7)
frame #9: PyEval_EvalCode + 0x19 (0x558f90a9e629 in /usr/bin/python2.7)
frame #10: <unknown function> + 0x12461f (0x558f90acf61f in /usr/bin/python2.7)
frame #11: PyRun_FileExFlags + 0x82 (0x558f90aca322 in /usr/bin/python2.7)
frame #12: PyRun_SimpleFileExFlags + 0x18d (0x558f90ac967d in /usr/bin/python2.7)
frame #13: Py_Main + 0x68b (0x558f90a781ab in /usr/bin/python2.7)
frame #14: __libc_start_main + 0xe7 (0x7f122ad02b97 in /lib/x86_64-linux-gnu/libc.so.6)
frame #15: _start + 0x2a (0x558f90a77a2a in /usr/bin/python2.7) | caffe2 | low | Critical |
397,360,423 | flutter | Please add automatic HashSet to Set type conversion to platform-channels. | Right now I have to convert from a `HashSet<T>` to an `ArrayList<T>` in Java and from a `List` back to a `Set` in dart.
Is it reasonable to add `Set` support to platform channels?
I am not an Objective-C programmer, but there is also `NSSet`, which seems to do the same thing.
Here are the references:
https://docs.oracle.com/javase/7/docs/api/java/util/Set.html
https://developer.apple.com/documentation/foundation/nsset
https://developer.apple.com/documentation/swift/set | c: new feature,framework,engine,P2,a: plugins,team-engine,triaged-engine | low | Minor |
397,396,961 | godot | iOS export configuration needs better validation | **Godot version:**
3.1 beta
**OS/device including version:**
macOS Mojave, Xcode
**Issue description:**
I've got a project that I'd like to export to iOS. The project can be found here: https://github.com/Krumelur/GodotTesting/tree/godot31_issue_24866
The export itself succeeds and I end up with an Xcode project file.
Deploying the project to a device fails with an error saying:
"App installation failed - No code signature found."
The reason is that no signing certificate is selected but *cannot* be selected. My assumption is that there is some Xcode project file compatibility issues. See video for details.
**Steps to reproduce:**
See videos for details. It will show exactly what I'm doing:
https://www.youtube.com/watch?v=5tXRo7uRPFE
* Open the project referenced above
* Export to iOS
* Try to deploy in Xcode
**Minimal reproduction project:**
see above | enhancement,platform:ios,topic:editor,usability | low | Critical |
397,425,552 | TypeScript | --noImplicitAny codefixes infer methods returning void instead of any | **TypeScript Version:** 3.3.0-dev.20190108
<!-- Search terms you tried before logging this (so others can find this issue more easily) -->
**Search Terms:** noimplicitany infer code fix codefix quick suggested return void any
**Code**
```ts
function curryThrough(callback) {
return callback("turtle");
}
```
**Expected behavior:**
`--noImplicitAny` suggested fix on the `callback` parameter:
```typescript
function curryThrough(callback: (arg0: string) => any) {
```
**Actual behavior:**
```typescript
function curryThrough(callback: (arg0: string) => void) {
```
**Related Issues:** #13243 _(parent tracking quick fixes)_
`void` seems like an odd default choice for the unknown return value of a method. If the type can't be inferred from usage, shouldn't it be `any`? | Suggestion,In Discussion | low | Minor |
397,429,475 | TypeScript | --noImplicitAny codefix inferring string for complex object | **TypeScript Version:** 3.3.0-dev.20190108
<!-- Search terms you tried before logging this (so others can find this issue more easily) -->
**Search Terms:** noimplicitany infer code fix codefix string object data
**Code**
```ts
function cleanup(data) {
if (Array.isArray(data)) {
return data.map(cleanup)
}
if (!data || typeof data !== 'object') return data
let keys = Object.keys(data)
if (keys.length <= 3 &&
data.name &&
(keys.length === 1 ||
keys.length === 3 && data.email && data.url ||
keys.length === 2 && (data.email || data.url))) {
data = unparsePerson(data)
}
return data
}
```
**Expected behavior:**
`--noImplicitAny` suggested fix on the `data` parameter: unclear what this should be. Perhaps a fancy type union containing an anonymous object type with fields such as `name` and `url`?
**Actual behavior:**
```typescript
function cleanup(data: string) {
```
**Related Issues:** #13243 _(parent tracking quick fixes)_
| Suggestion,Domain: Quick Fixes,Experience Enhancement | low | Minor |
397,444,961 | go | x/build/env: run more tests in js-wasm trybot mode | See #29632 wherein the build breaks due to js-wasm skipping too many tests on trybots.
We could just shard out the js-wasm trybot builder wider and enable more tests.
| Builders,NeedsInvestigation | low | Minor |
397,482,277 | TypeScript | Constructor property inheritance is not giving an error in ES2015+ target | **TypeScript Version:** 3.2.2
**Search Terms:** property inheritance constructor super
**Code**
```ts
abstract class A {
public names = ['A'];
}
class B extends A {
public names = [...super.names, 'B'];
}
const b = new B();
alert(b.names);
```
**Expected behavior:**
ES2015+ target: either TypeScript downlevels `super.names` in the child class to `this.names` which would make that code work, or it should give an error like it does in the ES5 version (check playground link).
Working ES2015 version:
```js
class A {
constructor() {
this.names = ['A'];
}
}
class B extends A {
constructor() {
super(...arguments);
this.names = [...this.names, 'B']; // `super` -> `this` so that this works as expected, but maybe not correct, not sure of the implications.
}
}
const b = new B();
alert(b.names);
```
So either that, or give a compile error. (ES5 target gives a compile error that has nothing to do with the actual error, so maybe even that should be sorted out)
**Actual behavior:**
Compiles fine under ES2015+ target, but gives a runtime error. (check stackblitz link)
**Playground Link:** https://www.typescriptlang.org/play/index.html#src=abstract%20class%20A%20%7B%0A%20%20public%20names%20%3D%20%5B'A'%5D%3B%0A%7D%0A%0Aclass%20B%20extends%20A%20%7B%0A%20%20public%20names%20%3D%20%5B...super.names%2C%20'B'%5D%3B%0A%7D%0A%0Aconst%20b%20%3D%20new%20B()%3B%0Aalert(b.names)%3B%0A
**Stackblitz Link:** https://stackblitz.com/edit/typescript-jcg479
**Related Issues:** I've tried, but couldn't really find anything similar. Forgive me if it has been reported already. | Bug | low | Critical |
397,570,013 | TypeScript | Path intellisense for other files besides js/ts | I would like to allow VScode intellisence can detect and show more file types when write import syntax.
for now when working with custom file type ex .vue file, editor only show js / ts file, .vue file is hidden.
my suggest is we have new setting:
`intellisence.suggest_file_types` and allow input custom file type want to load.
Like this we can control better.
| Suggestion,In Discussion | low | Major |
397,617,402 | flutter | IAP: Purchase a non-renewing subscription | c: new feature,p: in_app_purchase,package,team-ecosystem,P3,triaged-ecosystem | medium | Major |
|
397,637,587 | flutter | TextField shouldn't merge its semantics node into parent | ```dart
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: Foo(title: 'Flutter Demo Home Page'),
);
}
}
class Foo extends StatelessWidget {
Foo({this.title});
final String title;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(title),
),
body: Center(
child: Card(
child: Column(
children: [
Text('Hello'),
Text('Foo'),
TextField(
decoration: InputDecoration(
labelText: 'Enter Credit Card',
hintText: '1234',
),
),
],
),
),
),
);
}
}
```
Semantics Tree:
```
flutter: SemanticsNode#0
flutter: โ Rect.fromLTRB(0.0, 0.0, 750.0, 1334.0)
flutter: โ
flutter: โโSemanticsNode#1
flutter: โ Rect.fromLTRB(0.0, 0.0, 375.0, 667.0) scaled by 2.0x
flutter: โ textDirection: ltr
flutter: โ
flutter: โโSemanticsNode#2
flutter: โ Rect.fromLTRB(0.0, 0.0, 375.0, 667.0)
flutter: โ flags: scopesRoute
flutter: โ
flutter: โโSemanticsNode#4
flutter: โ โ Rect.fromLTRB(0.0, 0.0, 375.0, 76.0)
flutter: โ โ
flutter: โ โโSemanticsNode#5
flutter: โ Rect.fromLTRB(82.5, 36.0, 292.5, 60.0)
flutter: โ flags: isHeader
flutter: โ label: "Flutter Demo Home Page"
flutter: โ textDirection: ltr
flutter: โ
flutter: โโSemanticsNode#3
flutter: Rect.fromLTRB(0.0, 76.0, 375.0, 667.0)
flutter: actions: tap
flutter: flags: isTextField
flutter: label:
flutter: "Hello
flutter: Foo
flutter: Enter Credit Card"
flutter: textDirection: ltr
```
The textfield stuff ("Enter Credit Card" and SemanticsFlag.isTextField) should not be merged with the Text "Hallo" and "Foo" into one SemanticsNode. TextFields should always own their own SemanticsNodes. | a: text input,framework,f: material design,a: accessibility,customer: google,has reproducible steps,P2,workaround available,found in release: 3.7,team-design,triaged-design | low | Major |
397,700,600 | flutter | Camera document how to use ImageStream | First of all thank you so mush for adding access to the image stream in 0.2.8, I'm sure may people will be very happy about this.
I know the latest commit is fresh off the press, bit I would love to see a little more documentation on how to use the camera image stream (other than: "use: `cameraController.startImageStream(listener)` to process the images") | d: api docs,customer: crowd,p: camera,package,team-ecosystem,P2,triaged-ecosystem | low | Critical |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.