id
int64 393k
2.82B
| repo
stringclasses 68
values | title
stringlengths 1
936
| body
stringlengths 0
256k
β | labels
stringlengths 2
508
| priority
stringclasses 3
values | severity
stringclasses 3
values |
---|---|---|---|---|---|---|
377,029,804 | go | go/types: problem with alias type | The following code
```Go
package p
type F = func(T)
type T interface {
m(F)
}
type t struct{}
func (t) m(F) {}
var _ T = &t{}
```
doesn't type-check:
```
y.go:13:11: cannot use &(t literal) (value of type *t) as T value in variable declaration: wrong type for method m
```
but the code is correct (replacing F with func(T) everywhere works). | NeedsInvestigation,early-in-cycle | low | Major |
377,040,658 | rust | Matching on four consecutive bytes not optimized to matching on unaligned u32 | [Godbolt link](https://rust.godbolt.org/z/zmalWt)
Input
```rust
pub fn check(buf: &[u8]) -> bool {
if buf.len() < 4 {
return false;
}
match (buf[0] | 0x20, buf[1] | 0x20, buf[2], buf[3]) {
(b'a', b'b', b'+', b'-') => {
true
},
_ => {
false
}
}
}
pub fn check_case_sensitive(buf: &[u8]) -> bool {
if buf.len() < 4 {
return false;
}
match (buf[0], buf[1], buf[2], buf[3]) {
(b'a', b'b', b'+', b'-') => {
true
},
_ => {
false
}
}
}
```
Output
```asm
example::check:
cmp rsi, 3
ja .LBB0_2
xor eax, eax
ret
.LBB0_2:
mov al, byte ptr [rdi]
mov cl, byte ptr [rdi + 1]
or al, 32
or cl, 32
mov dl, byte ptr [rdi + 2]
mov sil, byte ptr [rdi + 3]
xor al, 97
xor cl, 98
or cl, al
xor dl, 43
or dl, cl
xor sil, 45
or sil, dl
sete al
ret
example::check_case_sensitive:
cmp rsi, 3
ja .LBB1_2
xor eax, eax
ret
.LBB1_2:
mov sil, byte ptr [rdi]
mov cl, byte ptr [rdi + 1]
mov dl, byte ptr [rdi + 2]
mov al, byte ptr [rdi + 3]
xor sil, 97
xor cl, 98
or cl, sil
xor dl, 43
or dl, cl
xor al, 45
or al, dl
sete al
ret
```
Expected there to be a single unaligned 32-bit load. Excepted the masking in the first function to be done on a 32-bit value using a 32-bit constant. Expected the comparison to be done with a 32-bit constant. | A-LLVM,I-slow,T-compiler | low | Minor |
377,059,190 | rust | Support for Moxie Virtual CPU | I would like to build a project that runs on the [Moxie Virtual CPU](http://moxielogic.org/blog/pages/about.html) which runs a PowerPC. Moxie has a complete GNU toolchain for C/C++ development. Furthermore there is already a target `powerpc-unknown-linux-gnu` which seems applicable to this situation.
My question is whether rust already supports Moxie and how I would go about using it. | A-LLVM,T-compiler,C-feature-request | low | Major |
377,089,533 | godot | Usability issues with AudioStreamPlayer3D attenuation model | From the few times I had to use `AudioStreamPlayer3D`, I faced several annoyances that I believe could get improvements, in particular with the attenuation model.
In frameworks and engines I used in the past, the attenuation model of a 3D sound has the following features:
- A minimum radius under which no attenuation is performed, in addition to the maximum radius above which the sound gets out of range
- Spheres are drawn in the 3D viewport to get an idea of the min/max distances
- Nice curves in the inspector showing how attenuation actually looks like over distance
On the other hand, in Godot:
- There is no minimum radius, and sound gets ALWAYS attenuated and muffled at any range, more or less. There is no way to decide "I want no low-pass attenuation within this distance, only start beyond it".
- There is no visualization of attenuation min/max radius in the main viewport
- There is no curves either, only numbers. Tweaking `Unit Size` to adapt the model to the distances in your game is counter-intuitive. For example it defaults to 1 (whatever that means), and all sounds get too muffled to my taste. I bumped it to 50 and it's still too muffled...
- There is no way to disable attenuation low-pass, other than forcing the cutoff frequency to 20500 Hz, which is also counter-intuitive.
Here are extra things that could enhance usability:
- When you get an attenuation model that suits you for one `AudioStreamPlayer3D`, you have to do it again for every single sound you want to play. A way to re-use models could be handy.
- Ability to have an attenuation-driven high-pass filter in addition to the low-pass filter. I came up with this need from experience, I've been creating audio series for a long time and when a character voice gets far from the "camera" it often sounds better when part of its low frequencies get cut off rather than its high frequencies (especially outside).
- From the same experience, I sometimes have to cross-fade two effect buses (one for `near`, one for `far`) for a sound that moves from near to really far away, but in Godot you can only output to one bus. How are we expected to do this without creating a bus for every sound? Do we always have to create two `AudioStreamPlayer3D` and play them both?
- On a stretch goal, Unity3D allows to configure the attenuation model very precisely, even allowing custom curves for each variable of the attenuation effect (volume, lowpass, highpass...). See https://docs.unity3d.com/Manual/class-AudioSource.html
I know some of this can be eventually scripted, but please at least give some consideration to the first part :) | enhancement,discussion,topic:editor,usability | medium | Major |
377,104,337 | opencv | HoughCircles: Unknown exception | I'm using [`4.0.0-beta`](https://opencv.org/releases.html) using the Java bindings. A user has reported the following exception when using the `HoughCircles` method:
```
Caused by: java.lang.Exception: unknown exception
at org.opencv.imgproc.Imgproc.HoughCircles_0(Native Method)
at org.opencv.imgproc.Imgproc.HoughCircles(Imgproc.java:1422)
...
```
This is the first and only time it happened so it's probably not reproducible since my code using Hough circles generally works:
```
public static Mat detectCircles(String imageFilePath, boolean showImage) throws InvocationTargetException, InterruptedException
{
val sourceImage = imread(imageFilePath, IMREAD_COLOR);
// Convert the image to gray
val gray = new Mat();
cvtColor(sourceImage, gray, COLOR_BGR2GRAY);
// Apply a median blur to reduce noise and avoid false circle detection
medianBlur(gray, gray, 5);
val circles = getCircles(gray);
drawRedCircles(sourceImage, circles);
if (showImage)
{
invokeAndWait(() -> imshow("Detected Circles", sourceImage));
waitKey();
}
return circles;
}
private static void drawRedCircles(Mat sourceImage, Mat circles)
{
for (var columnIndex = 0; columnIndex < circles.cols(); columnIndex++)
{
val rectangles = circles.get(0, columnIndex);
val center = new Point(round(rectangles[0]), round(rectangles[1]));
// circle center
circle(sourceImage, center, 1, new Scalar(0, 100, 100), 3, 8, 0);
// circle outline
val radius = (int) round(rectangles[2]);
circle(sourceImage, center, radius, new Scalar(255, 0, 255), 3, 8, 0);
}
}
private static Mat getCircles(Mat image)
{
val circles = new Mat();
HoughCircles(image, circles, HOUGH_GRADIENT, 1.0,
(double) image.rows() / 16,
100.0, 30.0, 10, 30);
return circles;
}
```
The platform is `Windows 10 64-bit`.
Can someone tell me more about this issue and if it's a bug which could be fixed?
Thank you! | priority: low,category: java bindings,incomplete,needs reproducer,needs investigation | low | Critical |
377,188,245 | go | proposal: cmd/go: add module repository health check tool | <!-- Please answer these questions before submitting your issue. Thanks! -->
### What version of Go are you using (`go version`)?
<pre>
$ go version
go version go1.11.1 linux/amd64
</pre>
### Does this issue reproduce with the latest release?
n/a
### What operating system and processor architecture are you using (`go env`)?
<details><summary><code>go env</code> Output</summary><br><pre>
$ go env
GOARCH="amd64"
GOBIN=""
GOCACHE="/home/myitcv/.cache/go-build"
GOEXE=""
GOFLAGS=""
GOHOSTARCH="amd64"
GOHOSTOS="linux"
GOOS="linux"
GOPATH="/home/myitcv/gostuff"
GOPROXY=""
GORACE=""
GOROOT="/home/myitcv/gos"
GOTMPDIR=""
GOTOOLDIR="/home/myitcv/gos/pkg/tool/linux_amd64"
GCCGO="gccgo"
CC="gcc"
CXX="g++"
CGO_ENABLED="1"
GOMOD=""
CGO_CFLAGS="-g -O2"
CGO_CPPFLAGS=""
CGO_CXXFLAGS="-g -O2"
CGO_FFLAGS="-g -O2"
CGO_LDFLAGS="-g -O2"
PKG_CONFIG="pkg-config"
GOGCCFLAGS="-fPIC -m64 -pthread -fmessage-length=0 -fdebug-prefix-map=/tmp/go-build925002674=/tmp/go-build -gno-record-gcc-switches"
</pre></details>
### Details
A number of issues and Slack and go-nuts questions boil down to a VCS repository being in some way "invalid" as far as modules are concerned. An incomplete list includes:
* `go.mod` for a `v >= 2` module not having a `/v2` suffix
* tags that are not semver compatible
* `go.mod` incomplete (i.e. the result of `go mod tidy` not committed)
* transitive dependencies leaking through to the main module because a dependency's `go.mod` is not complete
* Whether a version is compatible with Go pre 1.9.7 or 1.10.3
* ...
I think it might be useful to develop a tool that can be run against an arbitrary (remote) VCS repository that catches these sorts of problems, but also provides a summary of what is valid. A health check if you will.
This would be a tool for authors as well as consumers, because indicating (perhaps via one of those GitHub README badges) that your repository is module-safe is a good state and signal. Indeed in time this might be something that would sit well on godoc.org/similar, but in the short term a command line tool would have significant value.
These checks could of course be heavily cached (somewhere central).
This is a very rough idea, so just posting for thoughts/feedback:
```
$ modanalyse https://github.com/myitcv/terrible
Results for https://github.com/myitcv/terrible:
Semver issues:
0.0.1 is not compatible with Go modules; should be v0.0.1
0.0.1alpha is not a valid pre-release
Module completeness:
v0.0.3 is missing a go.sum
v0.0.4 has an incomplete go.mod
Invalid releases:
v2.0.0 invalid because go.mod does not end with /v2
Valid releases:
v0.0.2
v1.0.0
v2.0.1
```
cc @rsc @bcmills @thepudds @rogpeppe @mvdan | Proposal,GoCommand,modules | low | Critical |
377,189,651 | go | proposal: spec: language: make slices of constant strings constant if the indexes are constant | This will probably be assigned a Go2 label, although I think that it could be done during Go 1.
Consider multiline string constants:
```go
const multiline = `
This is a long text
that spans multiple lines
and then ends.
`
```
If you feed this text to a parser and it finds a problem on the first line ("This is a long text"), it will actually report that the error is on the *second* line because of the leading newline. One might think that this is easily solved by slicing the string:
```go
const multiline = `
This is a long text
that spans multiple lines
and then ends.
`[1:]
```
But this won't compile. The [Spec](https://golang.org/ref/spec#Slice_expressions) currently says:
>(β¦) For untyped string operands the result is a non-constant value of type string. (β¦)
Why not make it constant? After all, `len(multiline)` is constant.
* * *
There are other solutions to this. One could just use `multiline[1:]` everywhere where one doesn't want the leading newline. Or start "This is a long text" on the first line, but that hurts readability and "editability". Another solution is to make `multiline` a variable. The use case is pretty obscure and there isn't an immediate need for this, so this proposal is more about a question, why are slices (and indexing!) of constant strings not constant in the first place? | LanguageChange,Proposal,LanguageChangeReview | medium | Critical |
377,247,428 | vscode | [scss] rename not working for placeholder selectors | <!-- Please search existing issues to avoid creating duplicates. -->
<!-- Also please test using the latest insiders build to make sure your issue has not already been fixed: https://code.visualstudio.com/insiders/ -->
<!-- Use Help > Report Issue to prefill these. -->
- VSCode Version: 1.28.2
- OS Version: Windows 10
## Note: this only happens for `.scss` file types, as `.sass` is not supported by VSCode without extensions.
Steps to Reproduce:
1. Place cursor over sass placeholder selector
2. Initiate "Rename Symbol" command (through command palette, keyboard shortcuts, etc.)
3. Attempt to rename
After completion, the text editor will have renamed the variable throughout the document but removed the leading percent sign in the process. Even this can be dealt with by including a leading percent sign while renaming, it should be considered an issue because when the user does not take the effort to work around this quirk, the behavior of the selector is changed.
Example:
```sass
%foo {
...
}
.bar {
@extends %foo;
}
```
Attempting to rename `foo` to `foobar` results with this:
```sass
foobar {
...
}
.bar {
@extends foobar;
}
```
Rather than expected:
```sass
%foobar {
...
}
.bar {
@extends %foobar;
}
```
Does this issue occur when all extensions are disabled?: Yes
| feature-request,css-less-scss | low | Minor |
377,289,957 | TypeScript | Changing single-line JSDoc comments to multiline requires unnecessary edits | <!-- Please try to reproduce the issue with `typescript@next`. It may have already been fixed. -->
**TypeScript Version:** 3.1.3
<!-- Search terms you tried before logging this (so others can find this issue more easily) -->
**Search Terms:** jsdoc multiline
**Code**
```ts
/** |Foo */
```
1. Press <kbd>Enter</kbd> at the |
```ts
/**
* Foo |*/
```
2. Press <kbd>Enter</kbd> at the |
**Expected behavior:**
```ts
/**
* Foo
*/
```
**Actual behavior:**
```ts
/**
* Foo
* */
```
The leading `* ` has to be deleted now.
Empty JSDoc-Comments work:
```ts
/**| */
```
Press <kbd>Enter</kbd> at the |
```ts
/**
*
*/
``` | Suggestion,In Discussion,Domain: TSServer | low | Minor |
377,353,932 | flutter | [google_maps_flutter] How to customize infoWindowText in marker | I'm making a google maps for my android apps with custom infoWindowText.
I want to change the color, border, or add an image in the infoWindowText.
please help me | c: new feature,customer: crowd,p: maps,package,c: proposal,team-ecosystem,P3,triaged-ecosystem | medium | Critical |
377,440,465 | react | useCallback() invalidates too often in practice | This is related to https://github.com/facebook/react/issues/14092, https://github.com/facebook/react/issues/14066, https://github.com/reactjs/rfcs/issues/83, and some other issues.
The problem is that we often want to avoid invalidating a callback (e.g. to preserve shallow equality below or to avoid re-subscriptions in the effects). But if it depends on props or state, it's likely it'll invalidate too often. See https://github.com/facebook/react/issues/14092#issuecomment-435907249 for current workarounds.
`useReducer` doesn't suffer from this because the reducer is evaluated directly in the render phase. @sebmarkbage had an idea about giving `useCallback` similar semantics but it'll likely require complex implementation work. Seems like we'd have to do _something_ like this though.
I'm filing this just to acknowledge the issue exists, and to track further work on this. | Component: Hooks,React Core Team | high | Critical |
377,458,071 | godot | File browser type icon should be bigger on files that do not support a preview | **Godot version:**
3.1 alpha
**Issue description:**
The type icon for the files in the inspector is generally small. This is good for files that support a preview ( scripts, tscn, models) but for other type of files (saved animations) it is very small and requires more effort to be seen
**Proposal:**
When a file format does not support a preview, make the file type icon big and in the middle of the file icon.
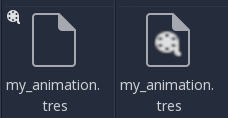
| enhancement,topic:editor,usability | low | Minor |
377,547,462 | go | cmd/compile: can't break on inlined function | ### What version of Go are you using (`go version`)?
<pre>
$ go version
go version devel +e72595ee0f Mon Nov 5 19:10:33 2018 +0000 linux/amd64
</pre>
(latest master)
### Does this issue reproduce with the latest release?
Yes.
### What operating system and processor architecture are you using (`go env`)?
<details><summary><code>go env</code> Output</summary><br><pre>
$ go env
GOARCH="amd64"
GOBIN=""
GOCACHE="/home/austin/.cache/go-build"
GOEXE=""
GOFLAGS=""
GOHOSTARCH="amd64"
GOHOSTOS="linux"
GOOS="linux"
GOPATH="/home/austin/r/go"
GOPROXY=""
GORACE=""
GOROOT="/home/austin/go.dev"
GOTMPDIR=""
GOTOOLDIR="/home/austin/go.dev/pkg/tool/linux_amd64"
GCCGO="/usr/bin/gccgo"
CC="gcc"
CXX="g++"
CGO_ENABLED="1"
GOMOD=""
CGO_CFLAGS="-g -O2"
CGO_CPPFLAGS=""
CGO_CXXFLAGS="-g -O2"
CGO_FFLAGS="-g -O2"
CGO_LDFLAGS="-g -O2"
PKG_CONFIG="pkg-config"
GOGCCFLAGS="-fPIC -m64 -pthread -fmessage-length=0 -fdebug-prefix-map=/tmp/go-build704555549=/tmp/go-build -gno-record-gcc-switches"
</pre></details>
### What did you do?
Attempt to set a breakpoint on an inlined function.
E.g., paste https://play.golang.org/p/rDXmoTUZKJb in to `bp.go` and run
```sh
go build bp.go
gdb ./bp
(gdb) br 'main.f'
Function "main.f" not defined.
(gdb) br 'main.main'
Breakpoint 1 at 0x455660: file /home/austin/go.dev/austin/bp.go, line 5.
```
### What did you expect to see?
I expected to be able to set a breakpoint on `main.f` like I could on `main.main`. In C/C++, this would cause gdb to set a breakpoint on every location the function was inlined to.
### What did you see instead?
`main.f` is completely missing from the debug info, so GDB is unable to set the breakpoint.
/cc @randall77 @thanm | NeedsInvestigation,compiler/runtime | low | Critical |
377,568,137 | TypeScript | Report `noImplicitThis` errors as suggestions when `noImplicitThis` is off | Title. Inspired by [this comment.](https://github.com/Microsoft/TypeScript/pull/28299#discussion_r230537698) | Suggestion,Committed,Domain: Error Messages | low | Critical |
377,580,587 | flutter | Support creation of add-to-app modules in Swift | `flutter create -t module` templates are missing the following:
- [x] The profile build configuration has an incorrect unique identifier reference to `Release.xcconfig` - copy paste issue.
- [ ] Swift language support. The templates currently only create ObjC variants, even if `-i swift` is passed on the command line. This is important when invoking the `make-host-app-editable` workflow.
/cc @matthew-carroll @mit-mit @xster | platform-ios,tool,a: existing-apps,P3,team-ios,triaged-ios | low | Major |
377,590,906 | go | x/build/revdial: race detected during execution of TestConnAgainstNetTest test | ### What version of Go are you using (`go version`)?
<pre>
$ go version
go version go1.11.2 darwin/amd64
</pre>
### Does this issue reproduce with the latest release?
Yes.
### What operating system and processor architecture are you using (`go env`)?
<details><summary><code>go env</code> Output</summary><br><pre>
$ go env
GOARCH="amd64"
GOBIN=""
GOCACHE="/Users/dmitshur/Library/Caches/go-build"
GOEXE=""
GOFLAGS=""
GOHOSTARCH="amd64"
GOHOSTOS="darwin"
GOOS="darwin"
GOPATH="/Users/dmitshur/go"
GOPROXY=""
GORACE=""
GOROOT="/usr/local/go"
GOTMPDIR=""
GOTOOLDIR="/usr/local/go/pkg/tool/darwin_amd64"
GCCGO="gccgo"
CC="clang"
CXX="clang++"
CGO_ENABLED="1"
GOMOD=""
CGO_CFLAGS="-g -O2"
CGO_CPPFLAGS=""
CGO_CXXFLAGS="-g -O2"
CGO_FFLAGS="-g -O2"
CGO_LDFLAGS="-g -O2"
PKG_CONFIG="pkg-config"
GOGCCFLAGS="-fPIC -m64 -pthread -fno-caret-diagnostics -Qunused-arguments -fmessage-length=0 -fdebug-prefix-map=/var/folders/3m/rg2zm24d1jg40wb48wr0hdjw00jwcj/T/go-build345908589=/tmp/go-build -gno-record-gcc-switches -fno-common"
</pre></details>
### What did you do?
```
$ go test -race golang.org/x/build/revdial
```
### What did you expect to see?
Tests to pass without races.
### What did you see instead?
Short version:
```
...
--- FAIL: TestConnAgainstNetTest (1.42s)
revdial_test.go:236: warning: the revdial's SetWriteDeadline support is not complete; some tests involving write deadlines known to be flaky
--- FAIL: TestConnAgainstNetTest/RacyWrite (0.15s)
testing.go:771: race detected during execution of test
--- FAIL: TestConnAgainstNetTest/FutureTimeout (0.63s)
testing.go:771: race detected during execution of test
testing.go:771: race detected during execution of test
FAIL
FAIL golang.org/x/build/revdial 1.485s
```
Full output:
<details><br>
```
$ go test -race golang.org/x/build/revdial
timeout for 2018-11-05 15:58:39.441634 -0500 EST m=+0.403776896
==================
WARNING: DATA RACE
Write at 0x00c0001a3180 by goroutine 47:
golang.org/x/build/revdial.(*conn).Write()
/Users/dmitshur/go/src/golang.org/x/build/revdial/revdial.go:438 +0x5cf
golang.org/x/net/nettest.testRacyWrite.func1()
/Users/dmitshur/go/src/golang.org/x/net/nettest/conntest.go:185 +0x124
Previous read at 0x00c0001a3180 by goroutine 46:
golang.org/x/build/revdial.(*conn).Write.func1()
/Users/dmitshur/go/src/golang.org/x/build/revdial/revdial.go:424 +0x1ab
Goroutine 47 (running) created at:
golang.org/x/net/nettest.testRacyWrite()
/Users/dmitshur/go/src/golang.org/x/net/nettest/conntest.go:179 +0x1d0
golang.org/x/net/nettest.timeoutWrapper()
/Users/dmitshur/go/src/golang.org/x/net/nettest/conntest.go:57 +0x2d8
golang.org/x/net/nettest.testConn.func4()
/Users/dmitshur/go/src/golang.org/x/net/nettest/conntest_go17.go:16 +0x57
testing.tRunner()
/usr/local/go/src/testing/testing.go:827 +0x162
Goroutine 46 (finished) created at:
golang.org/x/build/revdial.(*conn).Write()
/Users/dmitshur/go/src/golang.org/x/build/revdial/revdial.go:409 +0x3f9
golang.org/x/net/nettest.testRacyWrite.func1()
/Users/dmitshur/go/src/golang.org/x/net/nettest/conntest.go:185 +0x124
==================
timeout for 2018-11-05 15:58:39.441634 -0500 EST m=+0.403776896
timeout for 2018-11-05 15:58:39.442767 -0500 EST m=+0.404909548
==================
WARNING: DATA RACE
Read at 0x00c00010f000 by goroutine 24:
runtime.slicecopy()
/usr/local/go/src/runtime/slice.go:221 +0x0
bufio.(*Writer).Write()
/usr/local/go/src/bufio/bufio.go:619 +0x3ef
golang.org/x/build/revdial.writeFrame()
/Users/dmitshur/go/src/golang.org/x/build/revdial/revdial.go:224 +0x1f2
golang.org/x/build/revdial.(*conn).Write.func1()
/Users/dmitshur/go/src/golang.org/x/build/revdial/revdial.go:418 +0x129
Previous write at 0x00c00010f000 by goroutine 108:
runtime.slicecopy()
/usr/local/go/src/runtime/slice.go:221 +0x0
golang.org/x/net/nettest.testRacyWrite.func1()
/Users/dmitshur/go/src/golang.org/x/net/nettest/conntest.go:186 +0x185
Goroutine 24 (running) created at:
golang.org/x/build/revdial.(*conn).Write()
/Users/dmitshur/go/src/golang.org/x/build/revdial/revdial.go:409 +0x3f9
golang.org/x/net/nettest.testRacyWrite.func1()
/Users/dmitshur/go/src/golang.org/x/net/nettest/conntest.go:185 +0x124
Goroutine 108 (running) created at:
golang.org/x/net/nettest.testRacyWrite()
/Users/dmitshur/go/src/golang.org/x/net/nettest/conntest.go:179 +0x1d0
golang.org/x/net/nettest.timeoutWrapper()
/Users/dmitshur/go/src/golang.org/x/net/nettest/conntest.go:57 +0x2d8
golang.org/x/net/nettest.testConn.func4()
/Users/dmitshur/go/src/golang.org/x/net/nettest/conntest_go17.go:16 +0x57
testing.tRunner()
/usr/local/go/src/testing/testing.go:827 +0x162
==================
timeout for 2018-11-05 15:58:39.444006 -0500 EST m=+0.406148435
timeout for 2018-11-05 15:58:39.444006 -0500 EST m=+0.406148435
timeout for 2018-11-05 15:58:39.444006 -0500 EST m=+0.406148435
timeout for 2018-11-05 15:58:39.444006 -0500 EST m=+0.406148435
timeout for 2018-11-05 15:58:39.444006 -0500 EST m=+0.406148435
timeout for 2018-11-05 15:58:39.444006 -0500 EST m=+0.406148435
timeout for 2018-11-05 15:58:39.444006 -0500 EST m=+0.406148435
==================
WARNING: DATA RACE
Write at 0x00c000186960 by goroutine 24:
golang.org/x/build/revdial.(*conn).Write.func1()
/Users/dmitshur/go/src/golang.org/x/build/revdial/revdial.go:418 +0x14e
Previous write at 0x00c000186960 by goroutine 108:
golang.org/x/build/revdial.(*conn).Write()
/Users/dmitshur/go/src/golang.org/x/build/revdial/revdial.go:438 +0x5cf
golang.org/x/net/nettest.testRacyWrite.func1()
/Users/dmitshur/go/src/golang.org/x/net/nettest/conntest.go:185 +0x124
Goroutine 24 (running) created at:
golang.org/x/build/revdial.(*conn).Write()
/Users/dmitshur/go/src/golang.org/x/build/revdial/revdial.go:409 +0x3f9
golang.org/x/net/nettest.testRacyWrite.func1()
/Users/dmitshur/go/src/golang.org/x/net/nettest/conntest.go:185 +0x124
Goroutine 108 (running) created at:
golang.org/x/net/nettest.testRacyWrite()
/Users/dmitshur/go/src/golang.org/x/net/nettest/conntest.go:179 +0x1d0
golang.org/x/net/nettest.timeoutWrapper()
/Users/dmitshur/go/src/golang.org/x/net/nettest/conntest.go:57 +0x2d8
golang.org/x/net/nettest.testConn.func4()
/Users/dmitshur/go/src/golang.org/x/net/nettest/conntest_go17.go:16 +0x57
testing.tRunner()
/usr/local/go/src/testing/testing.go:827 +0x162
==================
timeout for 2018-11-05 15:58:39.444561 -0500 EST m=+0.406703891
timeout for 2018-11-05 15:58:39.444561 -0500 EST m=+0.406703891
timeout for 2018-11-05 15:58:39.444561 -0500 EST m=+0.406703891
timeout for 2018-11-05 15:58:39.445069 -0500 EST m=+0.407211799
timeout for 2018-11-05 15:58:39.445117 -0500 EST m=+0.407260406
timeout for 2018-11-05 15:58:39.445138 -0500 EST m=+0.407280723
timeout for 2018-11-05 15:58:39.445187 -0500 EST m=+0.407330012
timeout for 2018-11-05 15:58:39.445205 -0500 EST m=+0.407348041
timeout for 2018-11-05 15:58:39.445205 -0500 EST m=+0.407348041
timeout for 2018-11-05 15:58:39.445176 -0500 EST m=+0.407318477
timeout for 2018-11-05 15:58:39.445669 -0500 EST m=+0.407812242
timeout for 2018-11-05 15:58:39.445696 -0500 EST m=+0.407838575
timeout for 2018-11-05 15:58:39.445637 -0500 EST m=+0.407779472
timeout for 2018-11-05 15:58:39.446199 -0500 EST m=+0.408342267
timeout for 2018-11-05 15:58:39.446251 -0500 EST m=+0.408393828
timeout for 2018-11-05 15:58:39.446332 -0500 EST m=+0.408475397
timeout for 2018-11-05 15:58:39.446251 -0500 EST m=+0.408393828
timeout for 2018-11-05 15:58:39.446306 -0500 EST m=+0.408448531
timeout for 2018-11-05 15:58:39.446366 -0500 EST m=+0.408509135
timeout for 2018-11-05 15:58:39.446219 -0500 EST m=+0.408362057
timeout for 2018-11-05 15:58:39.446758 -0500 EST m=+0.408900843
timeout for 2018-11-05 15:58:39.446798 -0500 EST m=+0.408940682
timeout for 2018-11-05 15:58:39.446772 -0500 EST m=+0.408914957
timeout for 2018-11-05 15:58:39.447532 -0500 EST m=+0.409674689
timeout for 2018-11-05 15:58:39.44744 -0500 EST m=+0.409582945
timeout for 2018-11-05 15:58:39.447503 -0500 EST m=+0.409645799
timeout for 2018-11-05 15:58:39.44744 -0500 EST m=+0.409582945
timeout for 2018-11-05 15:58:39.447532 -0500 EST m=+0.409674689
timeout for 2018-11-05 15:58:39.447532 -0500 EST m=+0.409674689
timeout for 2018-11-05 15:58:39.447979 -0500 EST m=+0.410122241
timeout for 2018-11-05 15:58:39.448074 -0500 EST m=+0.410216593
timeout for 2018-11-05 15:58:39.448 -0500 EST m=+0.410143389
timeout for 2018-11-05 15:58:39.448 -0500 EST m=+0.410143389
timeout for 2018-11-05 15:58:39.448667 -0500 EST m=+0.410810038
timeout for 2018-11-05 15:58:39.448691 -0500 EST m=+0.410833582
timeout for 2018-11-05 15:58:39.448676 -0500 EST m=+0.410818726
timeout for 2018-11-05 15:58:39.448753 -0500 EST m=+0.410895914
timeout for 2018-11-05 15:58:39.448739 -0500 EST m=+0.410881477
timeout for 2018-11-05 15:58:39.448761 -0500 EST m=+0.410904194
timeout for 2018-11-05 15:58:39.449122 -0500 EST m=+0.411265046
timeout for 2018-11-05 15:58:39.449157 -0500 EST m=+0.411300159
timeout for 2018-11-05 15:58:39.449139 -0500 EST m=+0.411281508
timeout for 2018-11-05 15:58:39.449356 -0500 EST m=+0.411499172
timeout for 2018-11-05 15:58:39.449917 -0500 EST m=+0.412059733
timeout for 2018-11-05 15:58:39.4499 -0500 EST m=+0.412042847
timeout for 2018-11-05 15:58:39.449917 -0500 EST m=+0.412059733
timeout for 2018-11-05 15:58:39.449992 -0500 EST m=+0.412135309
timeout for 2018-11-05 15:58:39.449917 -0500 EST m=+0.412059733
timeout for 2018-11-05 15:58:39.450117 -0500 EST m=+0.412259487
timeout for 2018-11-05 15:58:39.4502 -0500 EST m=+0.412343373
timeout for 2018-11-05 15:58:39.450266 -0500 EST m=+0.412408489
timeout for 2018-11-05 15:58:39.450266 -0500 EST m=+0.412408489
timeout for 2018-11-05 15:58:39.450434 -0500 EST m=+0.412576422
timeout for 2018-11-05 15:58:39.451202 -0500 EST m=+0.413345225
timeout for 2018-11-05 15:58:39.451183 -0500 EST m=+0.413325977
timeout for 2018-11-05 15:58:39.451202 -0500 EST m=+0.413345225
timeout for 2018-11-05 15:58:39.451183 -0500 EST m=+0.413325977
timeout for 2018-11-05 15:58:39.451232 -0500 EST m=+0.413375392
timeout for 2018-11-05 15:58:39.451383 -0500 EST m=+0.413525837
timeout for 2018-11-05 15:58:39.451232 -0500 EST m=+0.413375392
timeout for 2018-11-05 15:58:39.451288 -0500 EST m=+0.413431312
timeout for 2018-11-05 15:58:39.451547 -0500 EST m=+0.413689824
timeout for 2018-11-05 15:58:39.451608 -0500 EST m=+0.413750689
timeout for 2018-11-05 15:58:39.452624 -0500 EST m=+0.414768787
timeout for 2018-11-05 15:58:39.452471 -0500 EST m=+0.414614222
timeout for 2018-11-05 15:58:39.452505 -0500 EST m=+0.414647592
timeout for 2018-11-05 15:58:39.452501 -0500 EST m=+0.414644034
timeout for 2018-11-05 15:58:39.452435 -0500 EST m=+0.414578162
timeout for 2018-11-05 15:58:39.452466 -0500 EST m=+0.414609198
timeout for 2018-11-05 15:58:39.452479 -0500 EST m=+0.414621883
timeout for 2018-11-05 15:58:39.452489 -0500 EST m=+0.414632255
timeout for 2018-11-05 15:58:39.452734 -0500 EST m=+0.414877141
timeout for 2018-11-05 15:58:39.452734 -0500 EST m=+0.414877141
timeout for 2018-11-05 15:58:39.453906 -0500 EST m=+0.416048771
timeout for 2018-11-05 15:58:39.453725 -0500 EST m=+0.415867568
timeout for 2018-11-05 15:58:39.453789 -0500 EST m=+0.415931723
timeout for 2018-11-05 15:58:39.453748 -0500 EST m=+0.415890741
timeout for 2018-11-05 15:58:39.453855 -0500 EST m=+0.415997645
timeout for 2018-11-05 15:58:39.453789 -0500 EST m=+0.415931723
timeout for 2018-11-05 15:58:39.453906 -0500 EST m=+0.416048771
timeout for 2018-11-05 15:58:39.454007 -0500 EST m=+0.416150156
timeout for 2018-11-05 15:58:39.453825 -0500 EST m=+0.415967825
timeout for 2018-11-05 15:58:39.453794 -0500 EST m=+0.415936614
timeout for 2018-11-05 15:58:39.455124 -0500 EST m=+0.417267200
timeout for 2018-11-05 15:58:39.455038 -0500 EST m=+0.417180963
timeout for 2018-11-05 15:58:39.455145 -0500 EST m=+0.417287764
timeout for 2018-11-05 15:58:39.455124 -0500 EST m=+0.417267200
timeout for 2018-11-05 15:58:39.45507 -0500 EST m=+0.417212417
timeout for 2018-11-05 15:58:39.455061 -0500 EST m=+0.417204317
timeout for 2018-11-05 15:58:39.455038 -0500 EST m=+0.417180963
timeout for 2018-11-05 15:58:39.45507 -0500 EST m=+0.417212417
timeout for 2018-11-05 15:58:39.455095 -0500 EST m=+0.417237847
timeout for 2018-11-05 15:58:39.455124 -0500 EST m=+0.417267200
timeout for 2018-11-05 15:58:39.456249 -0500 EST m=+0.418391414
timeout for 2018-11-05 15:58:39.456299 -0500 EST m=+0.418441567
timeout for 2018-11-05 15:58:39.456321 -0500 EST m=+0.418464055
timeout for 2018-11-05 15:58:39.456254 -0500 EST m=+0.418397381
timeout for 2018-11-05 15:58:39.456285 -0500 EST m=+0.418428069
timeout for 2018-11-05 15:58:39.456457 -0500 EST m=+0.418599763
timeout for 2018-11-05 15:58:39.456407 -0500 EST m=+0.418549795
timeout for 2018-11-05 15:58:39.456285 -0500 EST m=+0.418428069
timeout for 2018-11-05 15:58:39.45648 -0500 EST m=+0.418622739
timeout for 2018-11-05 15:58:39.456565 -0500 EST m=+0.418707935
timeout for 2018-11-05 15:58:39.457434 -0500 EST m=+0.419576960
timeout for 2018-11-05 15:58:39.45743 -0500 EST m=+0.419572759
timeout for 2018-11-05 15:58:39.45752 -0500 EST m=+0.419662704
timeout for 2018-11-05 15:58:39.457612 -0500 EST m=+0.419754814
timeout for 2018-11-05 15:58:39.457549 -0500 EST m=+0.419691648
timeout for 2018-11-05 15:58:39.457496 -0500 EST m=+0.419639131
timeout for 2018-11-05 15:58:39.457772 -0500 EST m=+0.419915223
timeout for 2018-11-05 15:58:39.457772 -0500 EST m=+0.419915223
timeout for 2018-11-05 15:58:39.457772 -0500 EST m=+0.419915223
timeout for 2018-11-05 15:58:39.457772 -0500 EST m=+0.419915223
timeout for 2018-11-05 15:58:39.45877 -0500 EST m=+0.420912788
timeout for 2018-11-05 15:58:39.45881 -0500 EST m=+0.420952574
timeout for 2018-11-05 15:58:39.458784 -0500 EST m=+0.420926685
timeout for 2018-11-05 15:58:39.458763 -0500 EST m=+0.420905424
timeout for 2018-11-05 15:58:39.458801 -0500 EST m=+0.420944348
timeout for 2018-11-05 15:58:39.45885 -0500 EST m=+0.420993290
timeout for 2018-11-05 15:58:39.458982 -0500 EST m=+0.421124987
timeout for 2018-11-05 15:58:39.458925 -0500 EST m=+0.421067510
timeout for 2018-11-05 15:58:39.458982 -0500 EST m=+0.421124987
timeout for 2018-11-05 15:58:39.458904 -0500 EST m=+0.421047263
timeout for 2018-11-05 15:58:39.459932 -0500 EST m=+0.422074564
timeout for 2018-11-05 15:58:39.459891 -0500 EST m=+0.422033627
timeout for 2018-11-05 15:58:39.459851 -0500 EST m=+0.421994007
timeout for 2018-11-05 15:58:39.459971 -0500 EST m=+0.422114025
timeout for 2018-11-05 15:58:39.460168 -0500 EST m=+0.422311244
timeout for 2018-11-05 15:58:39.460237 -0500 EST m=+0.422379523
timeout for 2018-11-05 15:58:39.460345 -0500 EST m=+0.422488316
timeout for 2018-11-05 15:58:39.460317 -0500 EST m=+0.422460419
timeout for 2018-11-05 15:58:39.460345 -0500 EST m=+0.422488316
timeout for 2018-11-05 15:58:39.46071 -0500 EST m=+0.422852588
timeout for 2018-11-05 15:58:39.461176 -0500 EST m=+0.423318521
timeout for 2018-11-05 15:58:39.461152 -0500 EST m=+0.423295401
timeout for 2018-11-05 15:58:39.4612 -0500 EST m=+0.423343140
timeout for 2018-11-05 15:58:39.461233 -0500 EST m=+0.423376324
timeout for 2018-11-05 15:58:39.461323 -0500 EST m=+0.423467373
timeout for 2018-11-05 15:58:39.461435 -0500 EST m=+0.423577720
timeout for 2018-11-05 15:58:39.461233 -0500 EST m=+0.423376324
timeout for 2018-11-05 15:58:39.461661 -0500 EST m=+0.423804107
timeout for 2018-11-05 15:58:39.461916 -0500 EST m=+0.424058988
timeout for 2018-11-05 15:58:39.462336 -0500 EST m=+0.424478886
timeout for 2018-11-05 15:58:39.462308 -0500 EST m=+0.424450976
timeout for 2018-11-05 15:58:39.462371 -0500 EST m=+0.424513610
timeout for 2018-11-05 15:58:39.462336 -0500 EST m=+0.424478886
timeout for 2018-11-05 15:58:39.462336 -0500 EST m=+0.424478886
timeout for 2018-11-05 15:58:39.462324 -0500 EST m=+0.424466809
timeout for 2018-11-05 15:58:39.462585 -0500 EST m=+0.424728116
timeout for 2018-11-05 15:58:39.462531 -0500 EST m=+0.424673444
timeout for 2018-11-05 15:58:39.462752 -0500 EST m=+0.424894814
timeout for 2018-11-05 15:58:39.46322 -0500 EST m=+0.425363167
timeout for 2018-11-05 15:58:39.463393 -0500 EST m=+0.425536100
timeout for 2018-11-05 15:58:39.463433 -0500 EST m=+0.425575739
timeout for 2018-11-05 15:58:39.463463 -0500 EST m=+0.425606364
timeout for 2018-11-05 15:58:39.463426 -0500 EST m=+0.425569031
timeout for 2018-11-05 15:58:39.463648 -0500 EST m=+0.425791234
timeout for 2018-11-05 15:58:39.463638 -0500 EST m=+0.425781366
timeout for 2018-11-05 15:58:39.463648 -0500 EST m=+0.425790724
timeout for 2018-11-05 15:58:39.463815 -0500 EST m=+0.425958419
timeout for 2018-11-05 15:58:39.46385 -0500 EST m=+0.425993321
timeout for 2018-11-05 15:58:39.464532 -0500 EST m=+0.426675082
timeout for 2018-11-05 15:58:39.46457 -0500 EST m=+0.426713425
timeout for 2018-11-05 15:58:39.46445 -0500 EST m=+0.426592756
timeout for 2018-11-05 15:58:39.464783 -0500 EST m=+0.426925453
timeout for 2018-11-05 15:58:39.464794 -0500 EST m=+0.426936825
timeout for 2018-11-05 15:58:39.464692 -0500 EST m=+0.426834845
timeout for 2018-11-05 15:58:39.464599 -0500 EST m=+0.426741823
timeout for 2018-11-05 15:58:39.464775 -0500 EST m=+0.426917602
timeout for 2018-11-05 15:58:39.464997 -0500 EST m=+0.427140381
timeout for 2018-11-05 15:58:39.465293 -0500 EST m=+0.427435833
timeout for 2018-11-05 15:58:39.465719 -0500 EST m=+0.427862134
timeout for 2018-11-05 15:58:39.465695 -0500 EST m=+0.427838055
timeout for 2018-11-05 15:58:39.465719 -0500 EST m=+0.427862134
timeout for 2018-11-05 15:58:39.465948 -0500 EST m=+0.428091222
timeout for 2018-11-05 15:58:39.465948 -0500 EST m=+0.428091222
timeout for 2018-11-05 15:58:39.465885 -0500 EST m=+0.428028898
timeout for 2018-11-05 15:58:39.46588 -0500 EST m=+0.428023369
timeout for 2018-11-05 15:58:39.466173 -0500 EST m=+0.428315666
timeout for 2018-11-05 15:58:39.466202 -0500 EST m=+0.428344873
timeout for 2018-11-05 15:58:39.46678 -0500 EST m=+0.428922963
timeout for 2018-11-05 15:58:39.466768 -0500 EST m=+0.428910443
timeout for 2018-11-05 15:58:39.467003 -0500 EST m=+0.429145498
timeout for 2018-11-05 15:58:39.46679 -0500 EST m=+0.428933032
timeout for 2018-11-05 15:58:39.46705 -0500 EST m=+0.429192423
timeout for 2018-11-05 15:58:39.46706 -0500 EST m=+0.429202910
timeout for 2018-11-05 15:58:39.467054 -0500 EST m=+0.429196583
timeout for 2018-11-05 15:58:39.467211 -0500 EST m=+0.429354082
timeout for 2018-11-05 15:58:39.467266 -0500 EST m=+0.429408928
timeout for 2018-11-05 15:58:39.467505 -0500 EST m=+0.429647866
timeout for 2018-11-05 15:58:39.468061 -0500 EST m=+0.430204340
timeout for 2018-11-05 15:58:39.468247 -0500 EST m=+0.430390211
timeout for 2018-11-05 15:58:39.468123 -0500 EST m=+0.430266208
timeout for 2018-11-05 15:58:39.468061 -0500 EST m=+0.430204340
timeout for 2018-11-05 15:58:39.468031 -0500 EST m=+0.430173434
timeout for 2018-11-05 15:58:39.46807 -0500 EST m=+0.430213429
timeout for 2018-11-05 15:58:39.468297 -0500 EST m=+0.430439686
timeout for 2018-11-05 15:58:39.468357 -0500 EST m=+0.430499915
timeout for 2018-11-05 15:58:39.468708 -0500 EST m=+0.430851422
timeout for 2018-11-05 15:58:39.468762 -0500 EST m=+0.430905083
timeout for 2018-11-05 15:58:39.469282 -0500 EST m=+0.431424919
timeout for 2018-11-05 15:58:39.469353 -0500 EST m=+0.431496294
timeout for 2018-11-05 15:58:39.469343 -0500 EST m=+0.431485665
timeout for 2018-11-05 15:58:39.469512 -0500 EST m=+0.431654732
timeout for 2018-11-05 15:58:39.469353 -0500 EST m=+0.431496294
timeout for 2018-11-05 15:58:39.469428 -0500 EST m=+0.431570939
timeout for 2018-11-05 15:58:39.469326 -0500 EST m=+0.431468885
timeout for 2018-11-05 15:58:39.469319 -0500 EST m=+0.431462037
timeout for 2018-11-05 15:58:39.470013 -0500 EST m=+0.432156393
timeout for 2018-11-05 15:58:39.470142 -0500 EST m=+0.432285388
timeout for 2018-11-05 15:58:39.470573 -0500 EST m=+0.432716219
timeout for 2018-11-05 15:58:39.470624 -0500 EST m=+0.432766503
timeout for 2018-11-05 15:58:39.470616 -0500 EST m=+0.432758937
timeout for 2018-11-05 15:58:39.470624 -0500 EST m=+0.432766503
timeout for 2018-11-05 15:58:39.470531 -0500 EST m=+0.432674078
timeout for 2018-11-05 15:58:39.470737 -0500 EST m=+0.432880031
timeout for 2018-11-05 15:58:39.470726 -0500 EST m=+0.432869145
timeout for 2018-11-05 15:58:39.470919 -0500 EST m=+0.433061773
timeout for 2018-11-05 15:58:39.471402 -0500 EST m=+0.433545338
timeout for 2018-11-05 15:58:39.471601 -0500 EST m=+0.433743712
timeout for 2018-11-05 15:58:39.471706 -0500 EST m=+0.433849151
timeout for 2018-11-05 15:58:39.471706 -0500 EST m=+0.433849151
timeout for 2018-11-05 15:58:39.471686 -0500 EST m=+0.433828953
timeout for 2018-11-05 15:58:39.471691 -0500 EST m=+0.433833506
timeout for 2018-11-05 15:58:39.471827 -0500 EST m=+0.433969802
timeout for 2018-11-05 15:58:39.471691 -0500 EST m=+0.433833506
timeout for 2018-11-05 15:58:39.471791 -0500 EST m=+0.433934080
timeout for 2018-11-05 15:58:39.472039 -0500 EST m=+0.434181911
timeout for 2018-11-05 15:58:39.472761 -0500 EST m=+0.434903715
timeout for 2018-11-05 15:58:39.472784 -0500 EST m=+0.434926707
timeout for 2018-11-05 15:58:39.472743 -0500 EST m=+0.434885666
timeout for 2018-11-05 15:58:39.472784 -0500 EST m=+0.434926707
timeout for 2018-11-05 15:58:39.472976 -0500 EST m=+0.435118532
timeout for 2018-11-05 15:58:39.473061 -0500 EST m=+0.435203455
timeout for 2018-11-05 15:58:39.473105 -0500 EST m=+0.435248105
timeout for 2018-11-05 15:58:39.473126 -0500 EST m=+0.435268891
timeout for 2018-11-05 15:58:39.473165 -0500 EST m=+0.435308253
timeout for 2018-11-05 15:58:39.473365 -0500 EST m=+0.435507936
timeout for 2018-11-05 15:58:39.473854 -0500 EST m=+0.435996712
timeout for 2018-11-05 15:58:39.473854 -0500 EST m=+0.435996712
timeout for 2018-11-05 15:58:39.473884 -0500 EST m=+0.436026701
timeout for 2018-11-05 15:58:39.474038 -0500 EST m=+0.436180865
timeout for 2018-11-05 15:58:39.47415 -0500 EST m=+0.436293357
timeout for 2018-11-05 15:58:39.474108 -0500 EST m=+0.436251131
timeout for 2018-11-05 15:58:39.47422 -0500 EST m=+0.436363311
timeout for 2018-11-05 15:58:39.47466 -0500 EST m=+0.436803124
timeout for 2018-11-05 15:58:39.474542 -0500 EST m=+0.436684722
timeout for 2018-11-05 15:58:39.475015 -0500 EST m=+0.437158148
timeout for 2018-11-05 15:58:39.474788 -0500 EST m=+0.436930511
timeout for 2018-11-05 15:58:39.475015 -0500 EST m=+0.437158148
timeout for 2018-11-05 15:58:39.474997 -0500 EST m=+0.437140287
timeout for 2018-11-05 15:58:39.475095 -0500 EST m=+0.437238292
timeout for 2018-11-05 15:58:39.475269 -0500 EST m=+0.437411603
timeout for 2018-11-05 15:58:39.475314 -0500 EST m=+0.437457380
timeout for 2018-11-05 15:58:39.47551 -0500 EST m=+0.437653246
timeout for 2018-11-05 15:58:39.476077 -0500 EST m=+0.438219432
timeout for 2018-11-05 15:58:39.476077 -0500 EST m=+0.438219432
timeout for 2018-11-05 15:58:39.476095 -0500 EST m=+0.438238071
timeout for 2018-11-05 15:58:39.476119 -0500 EST m=+0.438262374
timeout for 2018-11-05 15:58:39.476236 -0500 EST m=+0.438379073
timeout for 2018-11-05 15:58:39.476334 -0500 EST m=+0.438477062
timeout for 2018-11-05 15:58:39.476305 -0500 EST m=+0.438447996
timeout for 2018-11-05 15:58:39.476411 -0500 EST m=+0.438554251
timeout for 2018-11-05 15:58:39.47651 -0500 EST m=+0.438653350
timeout for 2018-11-05 15:58:39.47691 -0500 EST m=+0.439053132
timeout for 2018-11-05 15:58:39.477267 -0500 EST m=+0.439409586
timeout for 2018-11-05 15:58:39.477206 -0500 EST m=+0.439348812
timeout for 2018-11-05 15:58:39.477392 -0500 EST m=+0.439535231
timeout for 2018-11-05 15:58:39.477443 -0500 EST m=+0.439585634
timeout for 2018-11-05 15:58:39.477559 -0500 EST m=+0.439702243
timeout for 2018-11-05 15:58:39.47757 -0500 EST m=+0.439713022
timeout for 2018-11-05 15:58:39.477542 -0500 EST m=+0.439684801
timeout for 2018-11-05 15:58:39.477872 -0500 EST m=+0.440015207
timeout for 2018-11-05 15:58:39.478081 -0500 EST m=+0.440224080
timeout for 2018-11-05 15:58:39.478197 -0500 EST m=+0.440340100
timeout for 2018-11-05 15:58:39.478343 -0500 EST m=+0.440485948
timeout for 2018-11-05 15:58:39.4784 -0500 EST m=+0.440542950
timeout for 2018-11-05 15:58:39.478539 -0500 EST m=+0.440682232
timeout for 2018-11-05 15:58:39.47855 -0500 EST m=+0.440692534
timeout for 2018-11-05 15:58:39.478719 -0500 EST m=+0.440862324
timeout for 2018-11-05 15:58:39.478723 -0500 EST m=+0.440866357
timeout for 2018-11-05 15:58:39.479034 -0500 EST m=+0.441177222
timeout for 2018-11-05 15:58:39.479417 -0500 EST m=+0.441560095
timeout for 2018-11-05 15:58:39.479556 -0500 EST m=+0.441698604
timeout for 2018-11-05 15:58:39.479449 -0500 EST m=+0.441592013
timeout for 2018-11-05 15:58:39.479569 -0500 EST m=+0.441711923
timeout for 2018-11-05 15:58:39.47964 -0500 EST m=+0.441783062
timeout for 2018-11-05 15:58:39.47964 -0500 EST m=+0.441783062
timeout for 2018-11-05 15:58:39.47964 -0500 EST m=+0.441783062
timeout for 2018-11-05 15:58:39.479816 -0500 EST m=+0.441958521
timeout for 2018-11-05 15:58:39.479803 -0500 EST m=+0.441945859
timeout for 2018-11-05 15:58:39.48033 -0500 EST m=+0.442473431
timeout for 2018-11-05 15:58:39.48066 -0500 EST m=+0.442803221
timeout for 2018-11-05 15:58:39.480768 -0500 EST m=+0.442910498
timeout for 2018-11-05 15:58:39.480652 -0500 EST m=+0.442794992
timeout for 2018-11-05 15:58:39.480944 -0500 EST m=+0.443087334
timeout for 2018-11-05 15:58:39.480975 -0500 EST m=+0.443117963
timeout for 2018-11-05 15:58:39.481032 -0500 EST m=+0.443175092
timeout for 2018-11-05 15:58:39.480944 -0500 EST m=+0.443087334
timeout for 2018-11-05 15:58:39.481138 -0500 EST m=+0.443281026
timeout for 2018-11-05 15:58:39.481058 -0500 EST m=+0.443200727
timeout for 2018-11-05 15:58:39.481706 -0500 EST m=+0.443849051
timeout for 2018-11-05 15:58:39.481878 -0500 EST m=+0.444021346
timeout for 2018-11-05 15:58:39.481893 -0500 EST m=+0.444035588
timeout for 2018-11-05 15:58:39.481929 -0500 EST m=+0.444071805
timeout for 2018-11-05 15:58:39.482155 -0500 EST m=+0.444297760
timeout for 2018-11-05 15:58:39.482138 -0500 EST m=+0.444281265
timeout for 2018-11-05 15:58:39.482018 -0500 EST m=+0.444160502
timeout for 2018-11-05 15:58:39.482474 -0500 EST m=+0.444617226
timeout for 2018-11-05 15:58:39.482682 -0500 EST m=+0.444825029
timeout for 2018-11-05 15:58:39.482953 -0500 EST m=+0.445095724
timeout for 2018-11-05 15:58:39.482985 -0500 EST m=+0.445128283
timeout for 2018-11-05 15:58:39.482839 -0500 EST m=+0.444982028
timeout for 2018-11-05 15:58:39.483 -0500 EST m=+0.445142637
timeout for 2018-11-05 15:58:39.483232 -0500 EST m=+0.445375133
timeout for 2018-11-05 15:58:39.483289 -0500 EST m=+0.445432400
timeout for 2018-11-05 15:58:39.483352 -0500 EST m=+0.445494513
timeout for 2018-11-05 15:58:39.483514 -0500 EST m=+0.445656700
timeout for 2018-11-05 15:58:39.483794 -0500 EST m=+0.445937119
timeout for 2018-11-05 15:58:39.484047 -0500 EST m=+0.446190061
timeout for 2018-11-05 15:58:39.484424 -0500 EST m=+0.446566578
timeout for 2018-11-05 15:58:39.484392 -0500 EST m=+0.446534759
timeout for 2018-11-05 15:58:39.484424 -0500 EST m=+0.446566578
timeout for 2018-11-05 15:58:39.484583 -0500 EST m=+0.446725987
timeout for 2018-11-05 15:58:39.484621 -0500 EST m=+0.446764379
timeout for 2018-11-05 15:58:39.484622 -0500 EST m=+0.446764653
timeout for 2018-11-05 15:58:39.484789 -0500 EST m=+0.446931837
timeout for 2018-11-05 15:58:39.48469 -0500 EST m=+0.446832704
timeout for 2018-11-05 15:58:39.485146 -0500 EST m=+0.447289028
timeout for 2018-11-05 15:58:39.485423 -0500 EST m=+0.447565890
timeout for 2018-11-05 15:58:39.485584 -0500 EST m=+0.447726997
timeout for 2018-11-05 15:58:39.485574 -0500 EST m=+0.447716810
timeout for 2018-11-05 15:58:39.485782 -0500 EST m=+0.447924493
timeout for 2018-11-05 15:58:39.485829 -0500 EST m=+0.447972028
timeout for 2018-11-05 15:58:39.486076 -0500 EST m=+0.448219210
timeout for 2018-11-05 15:58:39.486145 -0500 EST m=+0.448288489
timeout for 2018-11-05 15:58:39.486192 -0500 EST m=+0.448335297
timeout for 2018-11-05 15:58:39.486213 -0500 EST m=+0.448356200
timeout for 2018-11-05 15:58:39.486666 -0500 EST m=+0.448809185
timeout for 2018-11-05 15:58:39.486679 -0500 EST m=+0.448822416
timeout for 2018-11-05 15:58:39.48681 -0500 EST m=+0.448953419
timeout for 2018-11-05 15:58:39.487101 -0500 EST m=+0.449243816
timeout for 2018-11-05 15:58:39.487231 -0500 EST m=+0.449374149
timeout for 2018-11-05 15:58:39.487269 -0500 EST m=+0.449411589
timeout for 2018-11-05 15:58:39.487607 -0500 EST m=+0.449749820
timeout for 2018-11-05 15:58:39.487531 -0500 EST m=+0.449673897
timeout for 2018-11-05 15:58:39.487652 -0500 EST m=+0.449794813
timeout for 2018-11-05 15:58:39.487837 -0500 EST m=+0.449979524
timeout for 2018-11-05 15:58:39.487757 -0500 EST m=+0.449899804
timeout for 2018-11-05 15:58:39.487957 -0500 EST m=+0.450099868
timeout for 2018-11-05 15:58:39.488405 -0500 EST m=+0.450547664
timeout for 2018-11-05 15:58:39.488623 -0500 EST m=+0.450765532
timeout for 2018-11-05 15:58:39.488664 -0500 EST m=+0.450807196
timeout for 2018-11-05 15:58:39.488779 -0500 EST m=+0.450922445
timeout for 2018-11-05 15:58:39.489086 -0500 EST m=+0.451228547
timeout for 2018-11-05 15:58:39.489086 -0500 EST m=+0.451228795
timeout for 2018-11-05 15:58:39.489304 -0500 EST m=+0.451446583
timeout for 2018-11-05 15:58:39.489387 -0500 EST m=+0.451529717
timeout for 2018-11-05 15:58:39.489108 -0500 EST m=+0.451251367
timeout for 2018-11-05 15:58:39.489532 -0500 EST m=+0.451674513
timeout for 2018-11-05 15:58:39.490028 -0500 EST m=+0.452171153
timeout for 2018-11-05 15:58:39.490031 -0500 EST m=+0.452173479
timeout for 2018-11-05 15:58:39.490005 -0500 EST m=+0.452148352
timeout for 2018-11-05 15:58:39.490457 -0500 EST m=+0.452600465
timeout for 2018-11-05 15:58:39.490457 -0500 EST m=+0.452600465
timeout for 2018-11-05 15:58:39.490822 -0500 EST m=+0.452964715
timeout for 2018-11-05 15:58:39.490457 -0500 EST m=+0.452600465
timeout for 2018-11-05 15:58:39.490909 -0500 EST m=+0.453052342
timeout for 2018-11-05 15:58:39.491002 -0500 EST m=+0.453144823
timeout for 2018-11-05 15:58:39.490942 -0500 EST m=+0.453085005
timeout for 2018-11-05 15:58:39.491392 -0500 EST m=+0.453535003
timeout for 2018-11-05 15:58:39.491575 -0500 EST m=+0.453718039
timeout for 2018-11-05 15:58:39.491498 -0500 EST m=+0.453640448
timeout for 2018-11-05 15:58:39.4918 -0500 EST m=+0.453942717
timeout for 2018-11-05 15:58:39.492 -0500 EST m=+0.454143126
timeout for 2018-11-05 15:58:39.492207 -0500 EST m=+0.454350227
timeout for 2018-11-05 15:58:39.492416 -0500 EST m=+0.454558742
timeout for 2018-11-05 15:58:39.492459 -0500 EST m=+0.454601939
timeout for 2018-11-05 15:58:39.492591 -0500 EST m=+0.454734203
timeout for 2018-11-05 15:58:39.492892 -0500 EST m=+0.455034791
timeout for 2018-11-05 15:58:39.492912 -0500 EST m=+0.455055109
timeout for 2018-11-05 15:58:39.493287 -0500 EST m=+0.455429864
timeout for 2018-11-05 15:58:39.493198 -0500 EST m=+0.455340838
timeout for 2018-11-05 15:58:39.493229 -0500 EST m=+0.455371554
timeout for 2018-11-05 15:58:39.493454 -0500 EST m=+0.455597069
timeout for 2018-11-05 15:58:39.493709 -0500 EST m=+0.455852031
timeout for 2018-11-05 15:58:39.49382 -0500 EST m=+0.455963216
timeout for 2018-11-05 15:58:39.494218 -0500 EST m=+0.456361182
timeout for 2018-11-05 15:58:39.494133 -0500 EST m=+0.456276321
timeout for 2018-11-05 15:58:39.494333 -0500 EST m=+0.456476190
timeout for 2018-11-05 15:58:39.494393 -0500 EST m=+0.456536248
timeout for 2018-11-05 15:58:39.494741 -0500 EST m=+0.456883950
timeout for 2018-11-05 15:58:39.494773 -0500 EST m=+0.456915687
timeout for 2018-11-05 15:58:39.494886 -0500 EST m=+0.457029331
timeout for 2018-11-05 15:58:39.494895 -0500 EST m=+0.457038118
timeout for 2018-11-05 15:58:39.495258 -0500 EST m=+0.457400764
timeout for 2018-11-05 15:58:39.495193 -0500 EST m=+0.457335519
timeout for 2018-11-05 15:58:39.495585 -0500 EST m=+0.457727539
timeout for 2018-11-05 15:58:39.495766 -0500 EST m=+0.457909180
timeout for 2018-11-05 15:58:39.495877 -0500 EST m=+0.458020448
timeout for 2018-11-05 15:58:39.495861 -0500 EST m=+0.458004073
timeout for 2018-11-05 15:58:39.496149 -0500 EST m=+0.458291686
timeout for 2018-11-05 15:58:39.496454 -0500 EST m=+0.458597148
timeout for 2018-11-05 15:58:39.496355 -0500 EST m=+0.458498215
timeout for 2018-11-05 15:58:39.496494 -0500 EST m=+0.458636530
timeout for 2018-11-05 15:58:39.496689 -0500 EST m=+0.458831935
timeout for 2018-11-05 15:58:39.49672 -0500 EST m=+0.458862515
timeout for 2018-11-05 15:58:39.496906 -0500 EST m=+0.459049299
timeout for 2018-11-05 15:58:39.497214 -0500 EST m=+0.459356855
timeout for 2018-11-05 15:58:39.497412 -0500 EST m=+0.459555114
timeout for 2018-11-05 15:58:39.497544 -0500 EST m=+0.459686448
timeout for 2018-11-05 15:58:39.497501 -0500 EST m=+0.459643906
timeout for 2018-11-05 15:58:39.497935 -0500 EST m=+0.460077958
timeout for 2018-11-05 15:58:39.498088 -0500 EST m=+0.460231086
timeout for 2018-11-05 15:58:39.498165 -0500 EST m=+0.460308095
timeout for 2018-11-05 15:58:39.498165 -0500 EST m=+0.460308095
timeout for 2018-11-05 15:58:39.498138 -0500 EST m=+0.460281378
timeout for 2018-11-05 15:58:39.498372 -0500 EST m=+0.460515483
timeout for 2018-11-05 15:58:39.498531 -0500 EST m=+0.460673705
timeout for 2018-11-05 15:58:39.498846 -0500 EST m=+0.460989252
timeout for 2018-11-05 15:58:39.498878 -0500 EST m=+0.461021194
timeout for 2018-11-05 15:58:39.49899 -0500 EST m=+0.461133133
timeout for 2018-11-05 15:58:39.499272 -0500 EST m=+0.461415151
timeout for 2018-11-05 15:58:39.49977 -0500 EST m=+0.461912929
timeout for 2018-11-05 15:58:39.499827 -0500 EST m=+0.461969824
timeout for 2018-11-05 15:58:39.499827 -0500 EST m=+0.461969824
timeout for 2018-11-05 15:58:39.499819 -0500 EST m=+0.461961490
timeout for 2018-11-05 15:58:39.49979 -0500 EST m=+0.461933329
timeout for 2018-11-05 15:58:39.500113 -0500 EST m=+0.462255879
timeout for 2018-11-05 15:58:39.500343 -0500 EST m=+0.462485579
timeout for 2018-11-05 15:58:39.500478 -0500 EST m=+0.462621443
timeout for 2018-11-05 15:58:39.500497 -0500 EST m=+0.462640143
timeout for 2018-11-05 15:58:39.500887 -0500 EST m=+0.463029685
timeout for 2018-11-05 15:58:39.50126 -0500 EST m=+0.463403246
timeout for 2018-11-05 15:58:39.501149 -0500 EST m=+0.463292071
timeout for 2018-11-05 15:58:39.50126 -0500 EST m=+0.463403246
timeout for 2018-11-05 15:58:39.501224 -0500 EST m=+0.463367061
timeout for 2018-11-05 15:58:39.50174 -0500 EST m=+0.463883298
timeout for 2018-11-05 15:58:39.501558 -0500 EST m=+0.463700512
timeout for 2018-11-05 15:58:39.501616 -0500 EST m=+0.463758512
timeout for 2018-11-05 15:58:39.50193 -0500 EST m=+0.464072854
timeout for 2018-11-05 15:58:39.501989 -0500 EST m=+0.464132041
timeout for 2018-11-05 15:58:39.502228 -0500 EST m=+0.464370672
timeout for 2018-11-05 15:58:39.502678 -0500 EST m=+0.464821425
timeout for 2018-11-05 15:58:39.502879 -0500 EST m=+0.465021968
timeout for 2018-11-05 15:58:39.502841 -0500 EST m=+0.464983975
timeout for 2018-11-05 15:58:39.502841 -0500 EST m=+0.464983975
timeout for 2018-11-05 15:58:39.503183 -0500 EST m=+0.465325839
timeout for 2018-11-05 15:58:39.503314 -0500 EST m=+0.465456714
timeout for 2018-11-05 15:58:39.503224 -0500 EST m=+0.465366542
timeout for 2018-11-05 15:58:39.50346 -0500 EST m=+0.465603073
timeout for 2018-11-05 15:58:39.503626 -0500 EST m=+0.465768985
timeout for 2018-11-05 15:58:39.503569 -0500 EST m=+0.465712374
timeout for 2018-11-05 15:58:39.50419 -0500 EST m=+0.466332837
timeout for 2018-11-05 15:58:39.504267 -0500 EST m=+0.466409940
timeout for 2018-11-05 15:58:39.50436 -0500 EST m=+0.466503147
timeout for 2018-11-05 15:58:39.50436 -0500 EST m=+0.466503147
timeout for 2018-11-05 15:58:39.504656 -0500 EST m=+0.466799314
timeout for 2018-11-05 15:58:39.504777 -0500 EST m=+0.466920518
timeout for 2018-11-05 15:58:39.504963 -0500 EST m=+0.467105858
timeout for 2018-11-05 15:58:39.50486 -0500 EST m=+0.467003276
timeout for 2018-11-05 15:58:39.505144 -0500 EST m=+0.467286658
timeout for 2018-11-05 15:58:39.50526 -0500 EST m=+0.467403163
timeout for 2018-11-05 15:58:39.50551 -0500 EST m=+0.467652864
timeout for 2018-11-05 15:58:39.505715 -0500 EST m=+0.467858051
timeout for 2018-11-05 15:58:39.505744 -0500 EST m=+0.467887105
timeout for 2018-11-05 15:58:39.50591 -0500 EST m=+0.468052620
timeout for 2018-11-05 15:58:39.506017 -0500 EST m=+0.468160316
timeout for 2018-11-05 15:58:39.506252 -0500 EST m=+0.468394578
timeout for 2018-11-05 15:58:39.506537 -0500 EST m=+0.468680445
timeout for 2018-11-05 15:58:39.506565 -0500 EST m=+0.468707504
timeout for 2018-11-05 15:58:39.506677 -0500 EST m=+0.468820037
timeout for 2018-11-05 15:58:39.506879 -0500 EST m=+0.469021652
timeout for 2018-11-05 15:58:39.506782 -0500 EST m=+0.468925373
timeout for 2018-11-05 15:58:39.507134 -0500 EST m=+0.469276768
timeout for 2018-11-05 15:58:39.507234 -0500 EST m=+0.469377029
timeout for 2018-11-05 15:58:39.507386 -0500 EST m=+0.469528842
timeout for 2018-11-05 15:58:39.507468 -0500 EST m=+0.469611401
timeout for 2018-11-05 15:58:39.507666 -0500 EST m=+0.469809023
timeout for 2018-11-05 15:58:39.508015 -0500 EST m=+0.470158264
timeout for 2018-11-05 15:58:39.508091 -0500 EST m=+0.470233754
timeout for 2018-11-05 15:58:39.50809 -0500 EST m=+0.470232575
timeout for 2018-11-05 15:58:39.508338 -0500 EST m=+0.470480811
timeout for 2018-11-05 15:58:39.50864 -0500 EST m=+0.470782866
timeout for 2018-11-05 15:58:39.508624 -0500 EST m=+0.470766927
timeout for 2018-11-05 15:58:39.508624 -0500 EST m=+0.470766927
timeout for 2018-11-05 15:58:39.508763 -0500 EST m=+0.470905687
timeout for 2018-11-05 15:58:39.509079 -0500 EST m=+0.471221786
timeout for 2018-11-05 15:58:39.509012 -0500 EST m=+0.471154958
timeout for 2018-11-05 15:58:39.509444 -0500 EST m=+0.471587203
timeout for 2018-11-05 15:58:39.5096 -0500 EST m=+0.471743013
timeout for 2018-11-05 15:58:39.509607 -0500 EST m=+0.471750191
timeout for 2018-11-05 15:58:39.509821 -0500 EST m=+0.471963520
timeout for 2018-11-05 15:58:39.510106 -0500 EST m=+0.472248540
timeout for 2018-11-05 15:58:39.510128 -0500 EST m=+0.472271261
timeout for 2018-11-05 15:58:39.510117 -0500 EST m=+0.472259843
timeout for 2018-11-05 15:58:39.51018 -0500 EST m=+0.472323075
timeout for 2018-11-05 15:58:39.510618 -0500 EST m=+0.472761487
timeout for 2018-11-05 15:58:39.510675 -0500 EST m=+0.472817876
timeout for 2018-11-05 15:58:39.511177 -0500 EST m=+0.473319563
timeout for 2018-11-05 15:58:39.51128 -0500 EST m=+0.473422519
timeout for 2018-11-05 15:58:39.511254 -0500 EST m=+0.473397242
timeout for 2018-11-05 15:58:39.51128 -0500 EST m=+0.473422519
timeout for 2018-11-05 15:58:39.51161 -0500 EST m=+0.473752653
timeout for 2018-11-05 15:58:39.511636 -0500 EST m=+0.473779052
timeout for 2018-11-05 15:58:39.511713 -0500 EST m=+0.473855573
timeout for 2018-11-05 15:58:39.511886 -0500 EST m=+0.474028623
timeout for 2018-11-05 15:58:39.51213 -0500 EST m=+0.474273261
timeout for 2018-11-05 15:58:39.512204 -0500 EST m=+0.474346852
timeout for 2018-11-05 15:58:39.512585 -0500 EST m=+0.474728306
timeout for 2018-11-05 15:58:39.51268 -0500 EST m=+0.474822860
timeout for 2018-11-05 15:58:39.512808 -0500 EST m=+0.474951222
timeout for 2018-11-05 15:58:39.512748 -0500 EST m=+0.474891037
timeout for 2018-11-05 15:58:39.513102 -0500 EST m=+0.475244521
timeout for 2018-11-05 15:58:39.513054 -0500 EST m=+0.475196529
timeout for 2018-11-05 15:58:39.513018 -0500 EST m=+0.475161287
timeout for 2018-11-05 15:58:39.51347 -0500 EST m=+0.475612658
timeout for 2018-11-05 15:58:39.513509 -0500 EST m=+0.475651666
timeout for 2018-11-05 15:58:39.513563 -0500 EST m=+0.475706347
timeout for 2018-11-05 15:58:39.513854 -0500 EST m=+0.475997074
timeout for 2018-11-05 15:58:39.51428 -0500 EST m=+0.476422618
timeout for 2018-11-05 15:58:39.514484 -0500 EST m=+0.476626608
timeout for 2018-11-05 15:58:39.514466 -0500 EST m=+0.476609075
timeout for 2018-11-05 15:58:39.514592 -0500 EST m=+0.476735308
timeout for 2018-11-05 15:58:39.514759 -0500 EST m=+0.476901856
timeout for 2018-11-05 15:58:39.514724 -0500 EST m=+0.476867292
timeout for 2018-11-05 15:58:39.514944 -0500 EST m=+0.477087105
timeout for 2018-11-05 15:58:39.515149 -0500 EST m=+0.477291965
timeout for 2018-11-05 15:58:39.515238 -0500 EST m=+0.477380608
timeout for 2018-11-05 15:58:39.515188 -0500 EST m=+0.477331083
timeout for 2018-11-05 15:58:39.515703 -0500 EST m=+0.477845573
timeout for 2018-11-05 15:58:39.516169 -0500 EST m=+0.478311607
timeout for 2018-11-05 15:58:39.516264 -0500 EST m=+0.478407028
timeout for 2018-11-05 15:58:39.516076 -0500 EST m=+0.478218998
timeout for 2018-11-05 15:58:39.516264 -0500 EST m=+0.478407028
timeout for 2018-11-05 15:58:39.516169 -0500 EST m=+0.478311607
timeout for 2018-11-05 15:58:39.516673 -0500 EST m=+0.478815673
timeout for 2018-11-05 15:58:39.516553 -0500 EST m=+0.478696066
timeout for 2018-11-05 15:58:39.516755 -0500 EST m=+0.478897591
timeout for 2018-11-05 15:58:39.516801 -0500 EST m=+0.478943502
timeout for 2018-11-05 15:58:39.517133 -0500 EST m=+0.479276031
timeout for 2018-11-05 15:58:39.517607 -0500 EST m=+0.479750329
timeout for 2018-11-05 15:58:39.517769 -0500 EST m=+0.479912284
timeout for 2018-11-05 15:58:39.517689 -0500 EST m=+0.479832245
timeout for 2018-11-05 15:58:39.517834 -0500 EST m=+0.479977157
timeout for 2018-11-05 15:58:39.517798 -0500 EST m=+0.479940552
timeout for 2018-11-05 15:58:39.518137 -0500 EST m=+0.480280355
timeout for 2018-11-05 15:58:39.518269 -0500 EST m=+0.480411603
timeout for 2018-11-05 15:58:39.518049 -0500 EST m=+0.480191727
timeout for 2018-11-05 15:58:39.518406 -0500 EST m=+0.480548696
timeout for 2018-11-05 15:58:39.518636 -0500 EST m=+0.480779351
timeout for 2018-11-05 15:58:39.519027 -0500 EST m=+0.481170345
timeout for 2018-11-05 15:58:39.519183 -0500 EST m=+0.481326198
timeout for 2018-11-05 15:58:39.519302 -0500 EST m=+0.481444590
timeout for 2018-11-05 15:58:39.519543 -0500 EST m=+0.481685908
timeout for 2018-11-05 15:58:39.519447 -0500 EST m=+0.481589656
timeout for 2018-11-05 15:58:39.519704 -0500 EST m=+0.481847237
timeout for 2018-11-05 15:58:39.519725 -0500 EST m=+0.481867685
timeout for 2018-11-05 15:58:39.519639 -0500 EST m=+0.481781837
timeout for 2018-11-05 15:58:39.52008 -0500 EST m=+0.482223084
timeout for 2018-11-05 15:58:39.520401 -0500 EST m=+0.482544224
timeout for 2018-11-05 15:58:39.520477 -0500 EST m=+0.482620006
timeout for 2018-11-05 15:58:39.520057 -0500 EST m=+0.482200085
timeout for 2018-11-05 15:58:39.521232 -0500 EST m=+0.483375251
timeout for 2018-11-05 15:58:39.521092 -0500 EST m=+0.483235329
timeout for 2018-11-05 15:58:39.521304 -0500 EST m=+0.483446760
timeout for 2018-11-05 15:58:39.52109 -0500 EST m=+0.483233049
timeout for 2018-11-05 15:58:39.521304 -0500 EST m=+0.483446760
timeout for 2018-11-05 15:58:39.52178 -0500 EST m=+0.483922889
timeout for 2018-11-05 15:58:39.521597 -0500 EST m=+0.483739990
timeout for 2018-11-05 15:58:39.521224 -0500 EST m=+0.483366950
timeout for 2018-11-05 15:58:39.521887 -0500 EST m=+0.484029592
timeout for 2018-11-05 15:58:39.522035 -0500 EST m=+0.484178250
timeout for 2018-11-05 15:58:39.522796 -0500 EST m=+0.484939359
timeout for 2018-11-05 15:58:39.522697 -0500 EST m=+0.484839973
timeout for 2018-11-05 15:58:39.522823 -0500 EST m=+0.484966341
timeout for 2018-11-05 15:58:39.522846 -0500 EST m=+0.484989241
timeout for 2018-11-05 15:58:39.522846 -0500 EST m=+0.484989241
timeout for 2018-11-05 15:58:39.523097 -0500 EST m=+0.485240373
timeout for 2018-11-05 15:58:39.523354 -0500 EST m=+0.485497492
timeout for 2018-11-05 15:58:39.523423 -0500 EST m=+0.485565516
timeout for 2018-11-05 15:58:39.523428 -0500 EST m=+0.485571056
timeout for 2018-11-05 15:58:39.523537 -0500 EST m=+0.485679778
timeout for 2018-11-05 15:58:39.524284 -0500 EST m=+0.486427005
timeout for 2018-11-05 15:58:39.524341 -0500 EST m=+0.486484078
timeout for 2018-11-05 15:58:39.524331 -0500 EST m=+0.486474203
timeout for 2018-11-05 15:58:39.52462 -0500 EST m=+0.486763434
timeout for 2018-11-05 15:58:39.524492 -0500 EST m=+0.486635226
timeout for 2018-11-05 15:58:39.524546 -0500 EST m=+0.486689468
timeout for 2018-11-05 15:58:39.524973 -0500 EST m=+0.487115647
timeout for 2018-11-05 15:58:39.524939 -0500 EST m=+0.487082339
timeout for 2018-11-05 15:58:39.525079 -0500 EST m=+0.487222370
timeout for 2018-11-05 15:58:39.524919 -0500 EST m=+0.487062310
timeout for 2018-11-05 15:58:39.525687 -0500 EST m=+0.487830442
timeout for 2018-11-05 15:58:39.525778 -0500 EST m=+0.487921128
timeout for 2018-11-05 15:58:39.525927 -0500 EST m=+0.488070446
timeout for 2018-11-05 15:58:39.525638 -0500 EST m=+0.487780681
timeout for 2018-11-05 15:58:39.52609 -0500 EST m=+0.488233051
timeout for 2018-11-05 15:58:39.52609 -0500 EST m=+0.488233051
timeout for 2018-11-05 15:58:39.526472 -0500 EST m=+0.488614630
timeout for 2018-11-05 15:58:39.526653 -0500 EST m=+0.488796426
timeout for 2018-11-05 15:58:39.526653 -0500 EST m=+0.488796426
timeout for 2018-11-05 15:58:39.526727 -0500 EST m=+0.488870059
timeout for 2018-11-05 15:58:39.527138 -0500 EST m=+0.489280827
timeout for 2018-11-05 15:58:39.527251 -0500 EST m=+0.489394391
timeout for 2018-11-05 15:58:39.527546 -0500 EST m=+0.489689072
timeout for 2018-11-05 15:58:39.527694 -0500 EST m=+0.489837253
timeout for 2018-11-05 15:58:39.527623 -0500 EST m=+0.489765812
timeout for 2018-11-05 15:58:39.527749 -0500 EST m=+0.489892396
timeout for 2018-11-05 15:58:39.528014 -0500 EST m=+0.490157132
timeout for 2018-11-05 15:58:39.528139 -0500 EST m=+0.490281762
timeout for 2018-11-05 15:58:39.52827 -0500 EST m=+0.490413256
timeout for 2018-11-05 15:58:39.528247 -0500 EST m=+0.490390146
timeout for 2018-11-05 15:58:39.528484 -0500 EST m=+0.490627082
timeout for 2018-11-05 15:58:39.528734 -0500 EST m=+0.490876832
timeout for 2018-11-05 15:58:39.528825 -0500 EST m=+0.490967994
timeout for 2018-11-05 15:58:39.529191 -0500 EST m=+0.491334049
timeout for 2018-11-05 15:58:39.529157 -0500 EST m=+0.491300362
timeout for 2018-11-05 15:58:39.52966 -0500 EST m=+0.491803166
timeout for 2018-11-05 15:58:39.529644 -0500 EST m=+0.491786977
timeout for 2018-11-05 15:58:39.529454 -0500 EST m=+0.491597297
timeout for 2018-11-05 15:58:39.529868 -0500 EST m=+0.492011102
timeout for 2018-11-05 15:58:39.529781 -0500 EST m=+0.491924217
timeout for 2018-11-05 15:58:39.529954 -0500 EST m=+0.492096515
timeout for 2018-11-05 15:58:39.530244 -0500 EST m=+0.492387016
timeout for 2018-11-05 15:58:39.530149 -0500 EST m=+0.492292071
timeout for 2018-11-05 15:58:39.530647 -0500 EST m=+0.492790469
timeout for 2018-11-05 15:58:39.530559 -0500 EST m=+0.492701605
timeout for 2018-11-05 15:58:39.531035 -0500 EST m=+0.493178233
timeout for 2018-11-05 15:58:39.531078 -0500 EST m=+0.493220990
timeout for 2018-11-05 15:58:39.531435 -0500 EST m=+0.493577931
timeout for 2018-11-05 15:58:39.531229 -0500 EST m=+0.493372494
timeout for 2018-11-05 15:58:39.531421 -0500 EST m=+0.493564085
timeout for 2018-11-05 15:58:39.531473 -0500 EST m=+0.493616429
timeout for 2018-11-05 15:58:39.531592 -0500 EST m=+0.493735165
timeout for 2018-11-05 15:58:39.531644 -0500 EST m=+0.493787090
timeout for 2018-11-05 15:58:39.531993 -0500 EST m=+0.494135694
timeout for 2018-11-05 15:58:39.531931 -0500 EST m=+0.494074177
timeout for 2018-11-05 15:58:39.532449 -0500 EST m=+0.494592227
timeout for 2018-11-05 15:58:39.532462 -0500 EST m=+0.494604533
timeout for 2018-11-05 15:58:39.532924 -0500 EST m=+0.495066750
timeout for 2018-11-05 15:58:39.533026 -0500 EST m=+0.495169419
timeout for 2018-11-05 15:58:39.533117 -0500 EST m=+0.495260155
timeout for 2018-11-05 15:58:39.533175 -0500 EST m=+0.495318099
timeout for 2018-11-05 15:58:39.533155 -0500 EST m=+0.495298365
timeout for 2018-11-05 15:58:39.533147 -0500 EST m=+0.495289967
timeout for 2018-11-05 15:58:39.53356 -0500 EST m=+0.495703420
timeout for 2018-11-05 15:58:39.533546 -0500 EST m=+0.495689216
timeout for 2018-11-05 15:58:39.533887 -0500 EST m=+0.496030025
timeout for 2018-11-05 15:58:39.53384 -0500 EST m=+0.495982728
timeout for 2018-11-05 15:58:39.534349 -0500 EST m=+0.496491871
timeout for 2018-11-05 15:58:39.534327 -0500 EST m=+0.496469983
timeout for 2018-11-05 15:58:39.534691 -0500 EST m=+0.496833903
timeout for 2018-11-05 15:58:39.534617 -0500 EST m=+0.496760212
timeout for 2018-11-05 15:58:39.53483 -0500 EST m=+0.496973268
timeout for 2018-11-05 15:58:39.535033 -0500 EST m=+0.497176355
timeout for 2018-11-05 15:58:39.534869 -0500 EST m=+0.497012511
timeout for 2018-11-05 15:58:39.535125 -0500 EST m=+0.497268128
timeout for 2018-11-05 15:58:39.535324 -0500 EST m=+0.497467485
timeout for 2018-11-05 15:58:39.535304 -0500 EST m=+0.497447196
timeout for 2018-11-05 15:58:39.535693 -0500 EST m=+0.497835669
timeout for 2018-11-05 15:58:39.535665 -0500 EST m=+0.497808271
timeout for 2018-11-05 15:58:39.536153 -0500 EST m=+0.498296463
timeout for 2018-11-05 15:58:39.536177 -0500 EST m=+0.498319805
timeout for 2018-11-05 15:58:39.536384 -0500 EST m=+0.498527227
timeout for 2018-11-05 15:58:39.536436 -0500 EST m=+0.498579335
timeout for 2018-11-05 15:58:39.536656 -0500 EST m=+0.498799472
timeout for 2018-11-05 15:58:39.536654 -0500 EST m=+0.498797182
timeout for 2018-11-05 15:58:39.536818 -0500 EST m=+0.498960694
timeout for 2018-11-05 15:58:39.536822 -0500 EST m=+0.498964758
timeout for 2018-11-05 15:58:39.537052 -0500 EST m=+0.499194806
timeout for 2018-11-05 15:58:39.537105 -0500 EST m=+0.499247926
timeout for 2018-11-05 15:58:39.537621 -0500 EST m=+0.499763627
timeout for 2018-11-05 15:58:39.537647 -0500 EST m=+0.499789959
timeout for 2018-11-05 15:58:39.53773 -0500 EST m=+0.499873255
timeout for 2018-11-05 15:58:39.537969 -0500 EST m=+0.500111540
timeout for 2018-11-05 15:58:39.538248 -0500 EST m=+0.500390526
timeout for 2018-11-05 15:58:39.538259 -0500 EST m=+0.500402375
timeout for 2018-11-05 15:58:39.538346 -0500 EST m=+0.500489502
timeout for 2018-11-05 15:58:39.538563 -0500 EST m=+0.500706346
timeout for 2018-11-05 15:58:39.538564 -0500 EST m=+0.500707323
timeout for 2018-11-05 15:58:39.538717 -0500 EST m=+0.500859796
timeout for 2018-11-05 15:58:39.539009 -0500 EST m=+0.501152351
timeout for 2018-11-05 15:58:39.539236 -0500 EST m=+0.501378941
timeout for 2018-11-05 15:58:39.539479 -0500 EST m=+0.501622350
timeout for 2018-11-05 15:58:39.539752 -0500 EST m=+0.501895184
timeout for 2018-11-05 15:58:39.539708 -0500 EST m=+0.501851221
timeout for 2018-11-05 15:58:39.539758 -0500 EST m=+0.501900847
timeout for 2018-11-05 15:58:39.539914 -0500 EST m=+0.502056789
timeout for 2018-11-05 15:58:39.539965 -0500 EST m=+0.502107585
timeout for 2018-11-05 15:58:39.540067 -0500 EST m=+0.502210215
timeout for 2018-11-05 15:58:39.540168 -0500 EST m=+0.502311233
timeout for 2018-11-05 15:58:39.540445 -0500 EST m=+0.502587581
timeout for 2018-11-05 15:58:39.54063 -0500 EST m=+0.502773037
timeout for 2018-11-05 15:58:39.54087 -0500 EST m=+0.503012546
timeout for 2018-11-05 15:58:39.541159 -0500 EST m=+0.503302007
timeout for 2018-11-05 15:58:39.541324 -0500 EST m=+0.503468681
timeout for 2018-11-05 15:58:39.541437 -0500 EST m=+0.503580070
timeout for 2018-11-05 15:58:39.541648 -0500 EST m=+0.503790867
timeout for 2018-11-05 15:58:39.541624 -0500 EST m=+0.503767272
timeout for 2018-11-05 15:58:39.541566 -0500 EST m=+0.503708621
timeout for 2018-11-05 15:58:39.541512 -0500 EST m=+0.503654737
timeout for 2018-11-05 15:58:39.542049 -0500 EST m=+0.504191957
timeout for 2018-11-05 15:58:39.542036 -0500 EST m=+0.504178833
timeout for 2018-11-05 15:58:39.542187 -0500 EST m=+0.504330450
timeout for 2018-11-05 15:58:39.542618 -0500 EST m=+0.504760568
timeout for 2018-11-05 15:58:39.542779 -0500 EST m=+0.504921832
timeout for 2018-11-05 15:58:39.543048 -0500 EST m=+0.505191078
timeout for 2018-11-05 15:58:39.543088 -0500 EST m=+0.505231419
timeout for 2018-11-05 15:58:39.543013 -0500 EST m=+0.505156162
timeout for 2018-11-05 15:58:39.543254 -0500 EST m=+0.505396698
timeout for 2018-11-05 15:58:39.543079 -0500 EST m=+0.505221560
timeout for 2018-11-05 15:58:39.543595 -0500 EST m=+0.505737691
timeout for 2018-11-05 15:58:39.543634 -0500 EST m=+0.505776662
timeout for 2018-11-05 15:58:39.543648 -0500 EST m=+0.505791403
timeout for 2018-11-05 15:58:39.544234 -0500 EST m=+0.506377450
timeout for 2018-11-05 15:58:39.544142 -0500 EST m=+0.506284684
timeout for 2018-11-05 15:58:39.544419 -0500 EST m=+0.506561907
timeout for 2018-11-05 15:58:39.544557 -0500 EST m=+0.506699907
timeout for 2018-11-05 15:58:39.544672 -0500 EST m=+0.506815189
timeout for 2018-11-05 15:58:39.544557 -0500 EST m=+0.506699907
timeout for 2018-11-05 15:58:39.544918 -0500 EST m=+0.507060727
timeout for 2018-11-05 15:58:39.545135 -0500 EST m=+0.507277614
timeout for 2018-11-05 15:58:39.54512 -0500 EST m=+0.507262766
timeout for 2018-11-05 15:58:39.545214 -0500 EST m=+0.507357310
timeout for 2018-11-05 15:58:39.545599 -0500 EST m=+0.507741818
timeout for 2018-11-05 15:58:39.5457 -0500 EST m=+0.507842944
timeout for 2018-11-05 15:58:39.54577 -0500 EST m=+0.507912641
timeout for 2018-11-05 15:58:39.546138 -0500 EST m=+0.508280746
timeout for 2018-11-05 15:58:39.546131 -0500 EST m=+0.508274505
timeout for 2018-11-05 15:58:39.546163 -0500 EST m=+0.508306119
timeout for 2018-11-05 15:58:39.546657 -0500 EST m=+0.508800350
timeout for 2018-11-05 15:58:39.546657 -0500 EST m=+0.508800350
timeout for 2018-11-05 15:58:39.546516 -0500 EST m=+0.508659366
timeout for 2018-11-05 15:58:39.546638 -0500 EST m=+0.508780611
timeout for 2018-11-05 15:58:39.546987 -0500 EST m=+0.509130406
timeout for 2018-11-05 15:58:39.547119 -0500 EST m=+0.509261960
timeout for 2018-11-05 15:58:39.547317 -0500 EST m=+0.509460367
timeout for 2018-11-05 15:58:39.547523 -0500 EST m=+0.509665912
timeout for 2018-11-05 15:58:39.547688 -0500 EST m=+0.509831351
timeout for 2018-11-05 15:58:39.547564 -0500 EST m=+0.509707491
timeout for 2018-11-05 15:58:39.548073 -0500 EST m=+0.510216337
timeout for 2018-11-05 15:58:39.548015 -0500 EST m=+0.510158215
timeout for 2018-11-05 15:58:39.548115 -0500 EST m=+0.510258066
timeout for 2018-11-05 15:58:39.548167 -0500 EST m=+0.510309659
timeout for 2018-11-05 15:58:39.5485 -0500 EST m=+0.510642970
timeout for 2018-11-05 15:58:39.548543 -0500 EST m=+0.510685666
timeout for 2018-11-05 15:58:39.548804 -0500 EST m=+0.510947080
timeout for 2018-11-05 15:58:39.549043 -0500 EST m=+0.511186031
timeout for 2018-11-05 15:58:39.549056 -0500 EST m=+0.511198999
timeout for 2018-11-05 15:58:39.549112 -0500 EST m=+0.511255135
timeout for 2018-11-05 15:58:39.549405 -0500 EST m=+0.511548153
timeout for 2018-11-05 15:58:39.549548 -0500 EST m=+0.511690948
timeout for 2018-11-05 15:58:39.549669 -0500 EST m=+0.511812150
timeout for 2018-11-05 15:58:39.549804 -0500 EST m=+0.511947180
timeout for 2018-11-05 15:58:39.550007 -0500 EST m=+0.512150225
timeout for 2018-11-05 15:58:39.549981 -0500 EST m=+0.512124073
timeout for 2018-11-05 15:58:39.550453 -0500 EST m=+0.512595998
timeout for 2018-11-05 15:58:39.550481 -0500 EST m=+0.512624346
timeout for 2018-11-05 15:58:39.55047 -0500 EST m=+0.512613343
timeout for 2018-11-05 15:58:39.550586 -0500 EST m=+0.512728773
timeout for 2018-11-05 15:58:39.55081 -0500 EST m=+0.512953448
timeout for 2018-11-05 15:58:39.55105 -0500 EST m=+0.513192983
timeout for 2018-11-05 15:58:39.551291 -0500 EST m=+0.513433840
timeout for 2018-11-05 15:58:39.551392 -0500 EST m=+0.513535031
timeout for 2018-11-05 15:58:39.551462 -0500 EST m=+0.513604875
timeout for 2018-11-05 15:58:39.551422 -0500 EST m=+0.513565310
timeout for 2018-11-05 15:58:39.551844 -0500 EST m=+0.513986937
timeout for 2018-11-05 15:58:39.551888 -0500 EST m=+0.514031533
timeout for 2018-11-05 15:58:39.552085 -0500 EST m=+0.514227939
timeout for 2018-11-05 15:58:39.552036 -0500 EST m=+0.514179485
timeout for 2018-11-05 15:58:39.552308 -0500 EST m=+0.514450694
timeout for 2018-11-05 15:58:39.552419 -0500 EST m=+0.514561566
timeout for 2018-11-05 15:58:39.552809 -0500 EST m=+0.514951575
timeout for 2018-11-05 15:58:39.552848 -0500 EST m=+0.514990806
timeout for 2018-11-05 15:58:39.553024 -0500 EST m=+0.515167290
timeout for 2018-11-05 15:58:39.553081 -0500 EST m=+0.515223730
timeout for 2018-11-05 15:58:39.553271 -0500 EST m=+0.515414222
timeout for 2018-11-05 15:58:39.553429 -0500 EST m=+0.515571620
timeout for 2018-11-05 15:58:39.553574 -0500 EST m=+0.515716859
timeout for 2018-11-05 15:58:39.553677 -0500 EST m=+0.515820459
timeout for 2018-11-05 15:58:39.553654 -0500 EST m=+0.515797037
timeout for 2018-11-05 15:58:39.553987 -0500 EST m=+0.516129799
timeout for 2018-11-05 15:58:39.55413 -0500 EST m=+0.516272566
timeout for 2018-11-05 15:58:39.55419 -0500 EST m=+0.516333147
timeout for 2018-11-05 15:58:39.554636 -0500 EST m=+0.516778913
timeout for 2018-11-05 15:58:39.554711 -0500 EST m=+0.516854436
timeout for 2018-11-05 15:58:39.554679 -0500 EST m=+0.516822058
timeout for 2018-11-05 15:58:39.55513 -0500 EST m=+0.517272873
timeout for 2018-11-05 15:58:39.555061 -0500 EST m=+0.517203748
timeout for 2018-11-05 15:58:39.555257 -0500 EST m=+0.517400297
timeout for 2018-11-05 15:58:39.555107 -0500 EST m=+0.517249646
timeout for 2018-11-05 15:58:39.555528 -0500 EST m=+0.517671131
timeout for 2018-11-05 15:58:39.555644 -0500 EST m=+0.517787243
timeout for 2018-11-05 15:58:39.55554 -0500 EST m=+0.517683370
timeout for 2018-11-05 15:58:39.55606 -0500 EST m=+0.518202731
timeout for 2018-11-05 15:58:39.556156 -0500 EST m=+0.518299512
timeout for 2018-11-05 15:58:39.556127 -0500 EST m=+0.518269961
timeout for 2018-11-05 15:58:39.556495 -0500 EST m=+0.518638332
timeout for 2018-11-05 15:58:39.556624 -0500 EST m=+0.518766679
timeout for 2018-11-05 15:58:39.556674 -0500 EST m=+0.518817464
timeout for 2018-11-05 15:58:39.556718 -0500 EST m=+0.518861227
timeout for 2018-11-05 15:58:39.556959 -0500 EST m=+0.519102113
timeout for 2018-11-05 15:58:39.557103 -0500 EST m=+0.519245612
timeout for 2018-11-05 15:58:39.557244 -0500 EST m=+0.519387098
timeout for 2018-11-05 15:58:39.557483 -0500 EST m=+0.519626050
timeout for 2018-11-05 15:58:39.557549 -0500 EST m=+0.519692533
timeout for 2018-11-05 15:58:39.557658 -0500 EST m=+0.519800656
timeout for 2018-11-05 15:58:39.557927 -0500 EST m=+0.520069934
timeout for 2018-11-05 15:58:39.558149 -0500 EST m=+0.520292304
timeout for 2018-11-05 15:58:39.558149 -0500 EST m=+0.520291842
timeout for 2018-11-05 15:58:39.558184 -0500 EST m=+0.520327246
timeout for 2018-11-05 15:58:39.558294 -0500 EST m=+0.520436838
timeout for 2018-11-05 15:58:39.558466 -0500 EST m=+0.520608774
timeout for 2018-11-05 15:58:39.558647 -0500 EST m=+0.520790377
timeout for 2018-11-05 15:58:39.558802 -0500 EST m=+0.520944753
timeout for 2018-11-05 15:58:39.559024 -0500 EST m=+0.521166673
timeout for 2018-11-05 15:58:39.559271 -0500 EST m=+0.521413898
timeout for 2018-11-05 15:58:39.559353 -0500 EST m=+0.521495931
timeout for 2018-11-05 15:58:39.559884 -0500 EST m=+0.522027072
timeout for 2018-11-05 15:58:39.559786 -0500 EST m=+0.521929261
timeout for 2018-11-05 15:58:39.559849 -0500 EST m=+0.521991753
timeout for 2018-11-05 15:58:39.559851 -0500 EST m=+0.521993657
timeout for 2018-11-05 15:58:39.56008 -0500 EST m=+0.522223073
timeout for 2018-11-05 15:58:39.560217 -0500 EST m=+0.522359983
timeout for 2018-11-05 15:58:39.560274 -0500 EST m=+0.522417292
timeout for 2018-11-05 15:58:39.560568 -0500 EST m=+0.522711221
timeout for 2018-11-05 15:58:39.560733 -0500 EST m=+0.522875641
timeout for 2018-11-05 15:58:39.560781 -0500 EST m=+0.522923615
timeout for 2018-11-05 15:58:39.561433 -0500 EST m=+0.523575681
timeout for 2018-11-05 15:58:39.561365 -0500 EST m=+0.523507759
timeout for 2018-11-05 15:58:39.561588 -0500 EST m=+0.523731345
timeout for 2018-11-05 15:58:39.561588 -0500 EST m=+0.523731345
timeout for 2018-11-05 15:58:39.561595 -0500 EST m=+0.523737777
timeout for 2018-11-05 15:58:39.561873 -0500 EST m=+0.524015784
timeout for 2018-11-05 15:58:39.561897 -0500 EST m=+0.524040379
timeout for 2018-11-05 15:58:39.562159 -0500 EST m=+0.524301866
timeout for 2018-11-05 15:58:39.56235 -0500 EST m=+0.524493169
timeout for 2018-11-05 15:58:39.562811 -0500 EST m=+0.524954260
timeout for 2018-11-05 15:58:39.562709 -0500 EST m=+0.524852062
timeout for 2018-11-05 15:58:39.562855 -0500 EST m=+0.524998532
timeout for 2018-11-05 15:58:39.563056 -0500 EST m=+0.525198605
timeout for 2018-11-05 15:58:39.562988 -0500 EST m=+0.525131218
timeout for 2018-11-05 15:58:39.56329 -0500 EST m=+0.525433227
timeout for 2018-11-05 15:58:39.563207 -0500 EST m=+0.525349938
timeout for 2018-11-05 15:58:39.563494 -0500 EST m=+0.525637470
timeout for 2018-11-05 15:58:39.563544 -0500 EST m=+0.525686692
timeout for 2018-11-05 15:58:39.564018 -0500 EST m=+0.526160989
timeout for 2018-11-05 15:58:39.564256 -0500 EST m=+0.526399207
timeout for 2018-11-05 15:58:39.564408 -0500 EST m=+0.526550829
timeout for 2018-11-05 15:58:39.564546 -0500 EST m=+0.526688832
timeout for 2018-11-05 15:58:39.564649 -0500 EST m=+0.526791955
timeout for 2018-11-05 15:58:39.564576 -0500 EST m=+0.526719236
timeout for 2018-11-05 15:58:39.564872 -0500 EST m=+0.527014640
timeout for 2018-11-05 15:58:39.564818 -0500 EST m=+0.526960817
timeout for 2018-11-05 15:58:39.565015 -0500 EST m=+0.527158067
timeout for 2018-11-05 15:58:39.565042 -0500 EST m=+0.527184989
timeout for 2018-11-05 15:58:39.565521 -0500 EST m=+0.527664520
timeout for 2018-11-05 15:58:39.56582 -0500 EST m=+0.527963041
timeout for 2018-11-05 15:58:39.565724 -0500 EST m=+0.527866948
timeout for 2018-11-05 15:58:39.566275 -0500 EST m=+0.528417949
timeout for 2018-11-05 15:58:39.566243 -0500 EST m=+0.528385745
timeout for 2018-11-05 15:58:39.566324 -0500 EST m=+0.528466766
timeout for 2018-11-05 15:58:39.566324 -0500 EST m=+0.528466766
timeout for 2018-11-05 15:58:39.566346 -0500 EST m=+0.528489480
timeout for 2018-11-05 15:58:39.566631 -0500 EST m=+0.528774141
timeout for 2018-11-05 15:58:39.566807 -0500 EST m=+0.528950530
timeout for 2018-11-05 15:58:39.567203 -0500 EST m=+0.529346127
timeout for 2018-11-05 15:58:39.56718 -0500 EST m=+0.529323153
timeout for 2018-11-05 15:58:39.56718 -0500 EST m=+0.529323153
timeout for 2018-11-05 15:58:39.567646 -0500 EST m=+0.529788809
timeout for 2018-11-05 15:58:39.567655 -0500 EST m=+0.529798415
timeout for 2018-11-05 15:58:39.567807 -0500 EST m=+0.529950277
timeout for 2018-11-05 15:58:39.56782 -0500 EST m=+0.529963201
timeout for 2018-11-05 15:58:39.568074 -0500 EST m=+0.530217397
timeout for 2018-11-05 15:58:39.568415 -0500 EST m=+0.530557629
timeout for 2018-11-05 15:58:39.568257 -0500 EST m=+0.530400194
timeout for 2018-11-05 15:58:39.568565 -0500 EST m=+0.530707900
timeout for 2018-11-05 15:58:39.568591 -0500 EST m=+0.530733756
timeout for 2018-11-05 15:58:39.568814 -0500 EST m=+0.530957299
timeout for 2018-11-05 15:58:39.569114 -0500 EST m=+0.531257012
timeout for 2018-11-05 15:58:39.569439 -0500 EST m=+0.531582384
timeout for 2018-11-05 15:58:39.569399 -0500 EST m=+0.531542153
timeout for 2018-11-05 15:58:39.569643 -0500 EST m=+0.531785689
timeout for 2018-11-05 15:58:39.569893 -0500 EST m=+0.532035945
timeout for 2018-11-05 15:58:39.569904 -0500 EST m=+0.532047382
timeout for 2018-11-05 15:58:39.570042 -0500 EST m=+0.532184998
timeout for 2018-11-05 15:58:39.570401 -0500 EST m=+0.532543694
timeout for 2018-11-05 15:58:39.570412 -0500 EST m=+0.532555106
timeout for 2018-11-05 15:58:39.570833 -0500 EST m=+0.532976138
timeout for 2018-11-05 15:58:39.570845 -0500 EST m=+0.532988468
timeout for 2018-11-05 15:58:39.571346 -0500 EST m=+0.533488977
timeout for 2018-11-05 15:58:39.571372 -0500 EST m=+0.533515403
timeout for 2018-11-05 15:58:39.571237 -0500 EST m=+0.533380352
timeout for 2018-11-05 15:58:39.571468 -0500 EST m=+0.533611544
timeout for 2018-11-05 15:58:39.57182 -0500 EST m=+0.533963169
timeout for 2018-11-05 15:58:39.571997 -0500 EST m=+0.534140487
timeout for 2018-11-05 15:58:39.572259 -0500 EST m=+0.534402067
timeout for 2018-11-05 15:58:39.572329 -0500 EST m=+0.534472432
timeout for 2018-11-05 15:58:39.572772 -0500 EST m=+0.534914898
timeout for 2018-11-05 15:58:39.57277 -0500 EST m=+0.534913539
timeout for 2018-11-05 15:58:39.572808 -0500 EST m=+0.534950862
timeout for 2018-11-05 15:58:39.573005 -0500 EST m=+0.535148579
timeout for 2018-11-05 15:58:39.57325 -0500 EST m=+0.535393454
timeout for 2018-11-05 15:58:39.573373 -0500 EST m=+0.535516133
timeout for 2018-11-05 15:58:39.573698 -0500 EST m=+0.535841068
timeout for 2018-11-05 15:58:39.573696 -0500 EST m=+0.535838936
timeout for 2018-11-05 15:58:39.574272 -0500 EST m=+0.536415289
timeout for 2018-11-05 15:58:39.574138 -0500 EST m=+0.536280755
timeout for 2018-11-05 15:58:39.574408 -0500 EST m=+0.536551292
timeout for 2018-11-05 15:58:39.574379 -0500 EST m=+0.536522092
timeout for 2018-11-05 15:58:39.574707 -0500 EST m=+0.536850348
timeout for 2018-11-05 15:58:39.574921 -0500 EST m=+0.537063623
timeout for 2018-11-05 15:58:39.575187 -0500 EST m=+0.537329989
timeout for 2018-11-05 15:58:39.575156 -0500 EST m=+0.537298810
timeout for 2018-11-05 15:58:39.575729 -0500 EST m=+0.537871559
timeout for 2018-11-05 15:58:39.575729 -0500 EST m=+0.537871559
timeout for 2018-11-05 15:58:39.575821 -0500 EST m=+0.537964302
timeout for 2018-11-05 15:58:39.575994 -0500 EST m=+0.538137238
timeout for 2018-11-05 15:58:39.57621 -0500 EST m=+0.538352755
timeout for 2018-11-05 15:58:39.576482 -0500 EST m=+0.538625544
timeout for 2018-11-05 15:58:39.576641 -0500 EST m=+0.538784448
timeout for 2018-11-05 15:58:39.577056 -0500 EST m=+0.539199126
timeout for 2018-11-05 15:58:39.57733 -0500 EST m=+0.539472776
timeout for 2018-11-05 15:58:39.577585 -0500 EST m=+0.539728501
timeout for 2018-11-05 15:58:39.577646 -0500 EST m=+0.539788710
timeout for 2018-11-05 15:58:39.57818 -0500 EST m=+0.540323530
timeout for 2018-11-05 15:58:39.578315 -0500 EST m=+0.540458332
timeout for 2018-11-05 15:58:39.578549 -0500 EST m=+0.540692355
timeout for 2018-11-05 15:58:39.57878 -0500 EST m=+0.540922880
timeout for 2018-11-05 15:58:39.579005 -0500 EST m=+0.541147995
timeout for 2018-11-05 15:58:39.58001 -0500 EST m=+0.542152790
timeout for 2018-11-05 15:58:39.57993 -0500 EST m=+0.542073207
timeout for 2018-11-05 15:58:39.579895 -0500 EST m=+0.542038220
timeout for 2018-11-05 15:58:39.580404 -0500 EST m=+0.542547439
timeout for 2018-11-05 15:58:39.580521 -0500 EST m=+0.542664347
timeout for 2018-11-05 15:58:39.581397 -0500 EST m=+0.543539832
timeout for 2018-11-05 15:58:39.581475 -0500 EST m=+0.543617730
timeout for 2018-11-05 15:58:39.581926 -0500 EST m=+0.544068664
timeout for 2018-11-05 15:58:39.581992 -0500 EST m=+0.544135148
timeout for 2018-11-05 15:58:39.583017 -0500 EST m=+0.545159994
timeout for 2018-11-05 15:58:39.583456 -0500 EST m=+0.545599424
timeout for 2018-11-05 15:58:39.584612 -0500 EST m=+0.546754825
timeout for 2018-11-05 15:58:39.585088 -0500 EST m=+0.547230852
timeout for 2018-11-05 15:58:39.586279 -0500 EST m=+0.548421805
timeout for 2018-11-05 15:58:39.586794 -0500 EST m=+0.548937095
timeout for 2018-11-05 15:58:39.587943 -0500 EST m=+0.550086535
timeout for 2018-11-05 15:58:39.797294 -0500 EST m=+0.759437361
==================
WARNING: DATA RACE
Write at 0x00c0000548f0 by goroutine 890:
golang.org/x/build/revdial.(*conn).Write.func1()
/Users/dmitshur/go/src/golang.org/x/build/revdial/revdial.go:418 +0x14e
Previous write at 0x00c0000548f0 by goroutine 879:
golang.org/x/build/revdial.(*conn).Write()
/Users/dmitshur/go/src/golang.org/x/build/revdial/revdial.go:438 +0x5cf
golang.org/x/net/nettest.testFutureTimeout.func2()
/Users/dmitshur/go/src/golang.org/x/net/nettest/conntest.go:297 +0xb2
Goroutine 890 (running) created at:
golang.org/x/build/revdial.(*conn).Write()
/Users/dmitshur/go/src/golang.org/x/build/revdial/revdial.go:409 +0x3f9
golang.org/x/net/nettest.testFutureTimeout.func2()
/Users/dmitshur/go/src/golang.org/x/net/nettest/conntest.go:297 +0xb2
Goroutine 879 (finished) created at:
golang.org/x/net/nettest.testFutureTimeout()
/Users/dmitshur/go/src/golang.org/x/net/nettest/conntest.go:293 +0x145
golang.org/x/net/nettest.timeoutWrapper()
/Users/dmitshur/go/src/golang.org/x/net/nettest/conntest.go:57 +0x2d8
golang.org/x/net/nettest.testConn.func9()
/Users/dmitshur/go/src/golang.org/x/net/nettest/conntest_go17.go:21 +0x57
testing.tRunner()
/usr/local/go/src/testing/testing.go:827 +0x162
==================
--- FAIL: TestConnAgainstNetTest (1.42s)
revdial_test.go:236: warning: the revdial's SetWriteDeadline support is not complete; some tests involving write deadlines known to be flaky
--- FAIL: TestConnAgainstNetTest/RacyWrite (0.15s)
testing.go:771: race detected during execution of test
--- FAIL: TestConnAgainstNetTest/FutureTimeout (0.63s)
testing.go:771: race detected during execution of test
testing.go:771: race detected during execution of test
FAIL
FAIL golang.org/x/build/revdial 1.485s
```
</details> | Testing,help wanted,Builders,NeedsInvestigation | low | Critical |
377,606,906 | scrcpy | Ctrl+Key shortcuts not working (Linux) | Just built from scrcpy-9999.ebuild. Ctrl+Key shortcuts not working, instead it acting like plain key is pressed (i.e. `Ctrl+V` entering **v** letter in input field). | input events,shortcuts | low | Major |
377,627,938 | angular | ActivatedRoute data loaded from other route when using same component for multiple lazy routes | # π bug report
### Affected Package
This issue happens with latest @angular/angular
### Is this a regression?
No
### Description
When I use the same component in multiple lazy-loaded routes, the `data` is loaded from the wrong route definition. Please see the stackblitz.
## π¬ Minimal Reproduction
https://stackblitz.com/edit/angular-issue-repro2-iiuvra?file=src%2Findex.html
## π Your Environment
**Angular Version:**
<pre><code>
Angular CLI: 7.0.4
Node: 8.12.0
OS: linux x64
Angular: 7.0.2
... animations, cdk, common, compiler, compiler-cli, core, forms
... http, language-service, material, platform-browser
... platform-browser-dynamic, router
Package Version
-----------------------------------------------------------
@angular-devkit/architect 0.10.4
@angular-devkit/build-angular 0.10.4
@angular-devkit/build-optimizer 0.10.4
@angular-devkit/build-webpack 0.10.4
@angular-devkit/core 7.0.4
@angular-devkit/schematics 7.0.4
@angular/cli 7.0.4
@ngtools/webpack 7.0.4
@schematics/angular 7.0.4
@schematics/update 0.10.4
rxjs 6.3.3
typescript 3.1.6
webpack 4.19.1
</code></pre>
**Anything else relevant?**
This also happens if I define `data` only on the second route, so the `data` returned is empty (`{}`)
[ActivatedRoute docs](https://angular.io/api/router/ActivatedRoute) says:
> Property | Description
> -- | --
> data: Observable<Data> | An observable of the static and resolved data of this route.
In my understanding, the `data` is for the current route, not for any other routes. | type: bug/fix,freq2: medium,area: router,state: confirmed,router: lazy loading,router: guards/resolvers,P3 | low | Critical |
377,629,200 | kubernetes | Allow forcing a put even when metadata.resourceVersion mismatches | atm when the resourceVersion mismatches kubectl pulls the latest and then retries, which is pointless since it just does the same request after
our usecase is similar, using the api to PUT the expected state, not caring for what the cluster thinks it is ... but we still get `metadata.resourceVersion: Invalid value` errors which is annoying and means we have to do a round-trip to get the latest version which might fail again if someone else updated the resource
... so support `?force=true` or `?noResourceVersion` to disable this | sig/api-machinery,lifecycle/frozen | medium | Critical |
377,633,784 | pytorch | caffe2: RuntimeError: [enforce fail at reshape_op.h:110] with Alexnet onnx test with cuda | ## π Bug
we are seeing an intermittent failure in the reshape_op when trying to run the EXAMPLE: END-TO-END ALEXNET FROM PYTORCH TO CAFFE2.
```
Traceback (most recent call last):
File "/tmp/test.py", line 35, in <module>
outputs = rep.run(np.random.randn(10, 3, 227, 227).astype(np.float32))
File "/opt/DL/pytorch/lib/python2.7/site-packages/caffe2/python/onnx/backend_rep.py", line 57, in run
self.workspace.RunNet(self.predict_net.name)
File "/opt/DL/pytorch/lib/python2.7/site-packages/caffe2/python/onnx/workspace.py", line 63, in f
return getattr(workspace, attr)(*args, **kwargs)
File "/opt/DL/pytorch/lib/python2.7/site-packages/caffe2/python/workspace.py", line 219, in RunNet
StringifyNetName(name), num_iter, allow_fail,
File "/opt/DL/pytorch/lib/python2.7/site-packages/caffe2/python/workspace.py", line 180, in CallWithExceptionIntercept
return func(*args, **kwargs)
RuntimeError: [enforce fail at reshape_op.h:110] total_size == size. 92160 vs -5680358544067434496. Argument `shape` does not agree with the input data. (92160 != -5680358544067434496)Error from operator:
input: "29" input: "36" output: "37" output: "OC2_DUMMY_1" name: "" type: "Reshape" device_option { device_type: 1 device_id: 0 }
```
## To Reproduce
Steps to reproduce the behavior:
Using the example code and the exported alexnet.onnx file run the following sample.
```
import onnx
import caffe2.python.onnx.backend as backend
import numpy as np
model = onnx.load("alexnet.onnx")
rep = backend.prepare(model, device="CUDA") # or "CPU"
outputs = rep.run(np.random.randn(10, 3, 224, 224).astype(np.float32))
print(outputs[0])
```
## Expected behavior
example code runs successfully every time.
## Environment
- PyTorch Version (e.g., 1.0): 1.0 rc1
- OS (e.g., Linux): RHEL 7.5 ppc64le
- How you installed PyTorch (`conda`, `pip`, source): Build from source
- Build command you used (if compiling from source): DEBUG=1 USE_OPENCV=1 python setup.py
- Python version: 2.7 or 3.6
- CUDA/cuDNN version: 10 7.31
- GPU models and configuration: v100
- Any other relevant information:
problem only occurs when use device = cuda, if device = cpu the issue is not see, seen on ppc64le unknown if other platforms are effected.
## Additional context
Checking that very large incorrect value, we see least significant
32-bits of the total size is correct (i.e. 0x16800, or 92160), but the
high order 32-bits are incorrect (should be 0x0, but contain data):
```
$ echo "obase=16; 2^64 -5680358544067434496" | bc
B12B500000016800
$ echo "ibase=16; 16800" | bc
92160
```
If we build Caffe2 to crash here, we find the cause of the problem seems
to be that the shape information copied back from the GPU is damaged,
for example in one run we see:
```
(gdb) print actual_new_shape
$6 = {<std::_Vector_base<long, std::allocator<long> >> = {
_M_impl = {<std::allocator<long>> = {<__gnu_cxx::new_allocator<long>> = {<No data fields>}, <No data fields>}, _M_start = 0x7ffdf836e930,
_M_finish = 0x7ffdf836e940, _M_end_of_storage = 0x7ffdf836e940}}, <No data fields>}
(gdb) x/4x 0x7ffdf836e930
0x7ffdf836e930: 0x0000000a 0x00000005 0x00002400 0x00000000
```
Here, 2 values (0x0a / 10, and 0x2400 / 9216) should have been copied in
from the GPU, but instead the most-significant 32-bits of the "10" value
have been overlayed with "0x05", resulting in a final apparent value of
0x0000 0005 0000 000a (21474836490).
Since the problem is intermittent, it's not clear whether the overwrite
is always happening (but mostly happens to be 0x0) or only happens
sometimes.
<!-- Add any other context about the problem here. -->
| caffe2 | low | Critical |
377,634,042 | TypeScript | React function-returning-component regression | **TypeScript Version:** 3.2.0-dev.20181103
**Code**
```ts
import React = require("react");
declare function F(): C;
class C extends React.Component<{ title?: string }> {}
<F title="My Title" />
```
**Expected behavior:**
No error, as in ts3.1.
**Actual behavior:**
```
src/a.tsx:4:2 - error TS2322: Type '{ title: string; }' is not assignable to type 'IntrinsicAttributes'.
Property 'title' does not exist on type 'IntrinsicAttributes'.
4 <F title="My Title" />
~
Found 1 error.
```
Discovered in `react-helmet/v4` and `react-flag-icon-css` on DefinitelyTyped. The `react-flag-icon-css` variation is more like:
```ts
import React = require("react");
declare class C extends React.PureComponent<{}> {}
declare const F: (props: {}) => C;
<C />; // OK
<F />; // Error
``` | Bug,Domain: JSX/TSX | low | Critical |
377,641,112 | TypeScript | [1.28.2] Refactor renaming class-method not renaming bind(this) assignments (Javascript) | - VSCode Version: 1.28.2
- OS Version: Windows 10
Steps to Reproduce:
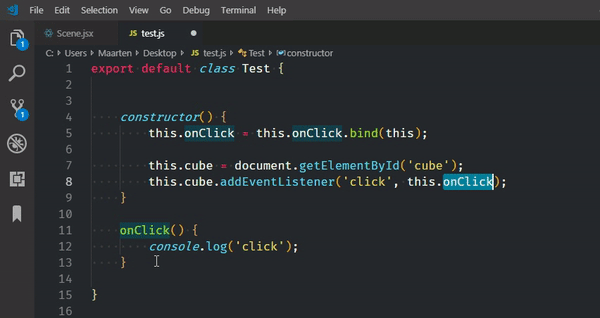
1. Refactor-rename class method (by hitting F2 to rename)
2. VS Code has not renamed the first methodname in this format:
`this.method = this.method.bind(this);`
Does this issue occur when all extensions are disabled?: Yes
| Bug,Domain: Refactorings | low | Minor |
377,642,397 | TypeScript | TypeScript doesn't allow event : CustomEvent in addEventListener | I'm using Visual Studio Code - Insiders v 1.29.0-insider
In my TypeScript project, I'm trying to write the following code:
buttonEl.addEventListener( 'myCustomEvent', ( event : CustomEvent ) => {
//do something
} );
The problem is that the CustomEvent type gives me the error shown below. If I replace CustomEvent with Event, then there is no error, but then I have difficulty getting event.detail out of the event listener.
"resource": "/c:/Users/me/Documents/app/file.ts",
"owner": "typescript",
"code": "2345",
"severity": 8,
"message": "Argument of type '(event: CustomEvent<any>) => void' is not assignable to parameter of type 'EventListenerOrEventListenerObject'.\n Type '(event: CustomEvent<any>) => void' is not assignable to type 'EventListener'.\n Types of parameters 'event' and 'evt' are incompatible.\n Type 'Event' is not assignable to type 'CustomEvent<any>'.\n Property 'detail' is missing in type 'Event'.",
"source": "ts",
"startLineNumber": 86,
"startColumn": 44,
"endLineNumber": 86,
"endColumn": 72
}
| Domain: lib.d.ts,Needs Investigation | high | Critical |
377,643,529 | TypeScript | The 'Convert to ES6 Module' in the TS language support has an aftereffect. | Issue Type: <b>Bug</b>
I chose the IntelliSense 'Convert to ES6 Module' at the import statement, then I generated it, but failed. If I don't convert, it runs fine. I think the convert of IntelliSense should be equivalent, at least the result should not cause a error.
```javascript
var commandExists = require("command-exists").sync
console.log(commandExists('C://MinGW//bin//g++.exe'))
```
VS Code version: Code 1.28.2 (7f3ce96ff4729c91352ae6def877e59c561f4850, 2018-10-17T00:23:51.859Z)
OS version: Windows_NT x64 10.0.17134
<details>
<summary>System Info</summary>
|Item|Value|
|---|---|
|CPUs|Intel(R) Core(TM) i5-5200U CPU @ 2.20GHz (4 x 2195)|
|GPU Status|2d_canvas: enabled<br>checker_imaging: disabled_off<br>flash_3d: enabled<br>flash_stage3d: enabled<br>flash_stage3d_baseline: enabled<br>gpu_compositing: enabled<br>multiple_raster_threads: enabled_on<br>native_gpu_memory_buffers: disabled_software<br>rasterization: enabled<br>video_decode: enabled<br>video_encode: enabled<br>webgl: enabled<br>webgl2: enabled|
|Memory (System)|3.92GB (0.59GB free)|
|Process Argv||
|Screen Reader|no|
|VM|0%|
</details>Extensions: none
<!-- generated by issue reporter --> | Suggestion,Awaiting More Feedback | low | Critical |
377,656,596 | TypeScript | Error message on JSX in .ts file should suggest using .tsx | **TypeScript Version:** 3.2.0-dev.20181103
**Code**
```ts
<div></div>;
```
**Expected behavior:**
Error message tells me to change the file extension to `.tsx`.
**Actual behavior:**
Error message says `Cannot find name 'div'.`, which makes me think I have to install `@types/div` or something. | Suggestion,Domain: Error Messages,Experience Enhancement | low | Critical |
377,661,075 | flutter | Can't determine the location of a long/double tap | If a `DoubleTapGestureRecognizer` won in the gesture arena, there's no equivalent of a forced flushing of a `onTapDown` like for `TapGestureRecognizer` to get the location of the double tap.
Similarly, `LongPressGestureRecognizer`'s `onLongPressUp` doesn't provide a location like `TapGestureRecognizer.onTapUp` | c: new feature,framework,f: gestures,c: proposal,P2,team-framework,triaged-framework | low | Minor |
377,671,740 | go | cmd/cgo: []byte argument has Go pointer to Go pointer | With Go 1.11 and HEAD: `go version devel +a2a3dd00c9 Thu Sep 13 09:52:57 2018 +0000 darwin/amd64`, the following program:
```
package main
// void f(void* ptr) {}
import "C"
import (
"compress/gzip"
"unsafe"
)
type cgoWriter struct{}
func (cgoWriter) Write(p []byte) (int, error) {
ptr := unsafe.Pointer(&p[0])
C.f(ptr)
return 0, nil
}
func main() {
w := cgoWriter{}
gzw := gzip.NewWriter(w)
gzw.Close() // calls cgoWriter.Write
}
```
Panics:
```
panic: runtime error: cgo argument has Go pointer to Go pointer
goroutine 1 [running]:
main.cgoWriter.Write.func1(0xc00015e020)
/Users/crawshaw/junk.go:15 +0x48
main.cgoWriter.Write(0xc00015e020, 0x1, 0xf8, 0xc0000b1e50, 0x4027ff1, 0x40dad60)
/Users/crawshaw/junk.go:15 +0x35
compress/flate.(*huffmanBitWriter).write(0xc00015e000, 0xc00015e020, 0x1, 0xf8)
/Users/crawshaw/go/go/src/compress/flate/huffman_bit_writer.go:136 +0x5f
compress/flate.(*huffmanBitWriter).flush(0xc00015e000)
/Users/crawshaw/go/go/src/compress/flate/huffman_bit_writer.go:128 +0xb7
compress/flate.(*huffmanBitWriter).writeStoredHeader(0xc00015e000, 0x0, 0x1)
/Users/crawshaw/go/go/src/compress/flate/huffman_bit_writer.go:412 +0x63
compress/flate.(*compressor).close(0xc0000ba000, 0x0, 0xc0000a62b0)
/Users/crawshaw/go/go/src/compress/flate/deflate.go:647 +0x83
compress/flate.(*Writer).Close(0xc0000ba000, 0x0, 0x0)
/Users/crawshaw/go/go/src/compress/flate/deflate.go:729 +0x2d
compress/gzip.(*Writer).Close(0xc0000a6210, 0x419bf30, 0xc0000a6210)
/Users/crawshaw/go/go/src/compress/gzip/gzip.go:242 +0x6e
main.main()
/Users/crawshaw/junk.go:22 +0x47
```
I suspect (but cannot yet show that) this is related to the fact that gzip.Writer is passing a []byte to the Write method made out of an array field of the gzip.Writer struct. | NeedsFix | low | Critical |
377,681,155 | create-react-app | Svg will crack when import with ReactComponent | I have several needs to hover a button and change color of the svg path stroke/fill
It will work perfectly most of time I use it as a component can assign a classname.
While sometimes the svg image will crack and I sure it is complete when I use it as <img src=...>
I think it is a bug, thanks for help
FYI svg source : https://reurl.cc/8yAkX
> Import {ReactComopnent as Graph } from '..../.svg'
>
> render() ....
> <div> < Graph/> </div>
npm 6.4.1
react 16.6.0
---------------------------------------------------------------------------------------------
Thanks for your patient :)
I finally get a simple one.
Here is the code :
```
import React, { Component } from 'react';
import './App.css';
import {ReactComponent as A }γfrom './A.svg'
import {ReactComponent as B }γfrom './B.svg'
class App extends Component {
render() {
return (
<div className="App">
<A/>
<B/>
</div>
);
}
}
export default App;
```
and FYI here is those 2 svg
https://reurl.cc/ZGlzg
https://reurl.cc/gWnEV
btw, you might need set background color to see it clearly.
This is how it happened :
as long as I use them in the same time, one specific svg will crack.
In this situation, B will become an incomplete image...
like [this](https://reurl.cc/6abRd) :
Not for every svg. I try to edit svg like delete <defs> and <mask>
It works only for another svg..., but not for every svg files.
Thanks for help | issue: needs investigation | medium | Critical |
377,681,197 | go | proposal: spec: express pointer/struct/slice/map/array types as possibly-const interface types | I propose that it be possible to express pointer, struct, slice, map, and array types as interface types. This issue is an informal description of the idea to see what people think.
Expressing one of these types as an interface type will be written as though the non-interface type were embedded in the interface type, as in `type TI interface { T }`, or in expanded form as `type TS interface { struct { f1 int; f2 string } }`. An interface of this form may be used exactly as the embedded type, except that all operations on the type are implemented as method calls on the embedded type. For example, one may write
```Go
type S struct {
f1 int
f2 string
}
type TS interface {
S
}
func F(ts TS) int {
ts.f1++
return ts.f1 + len(ts.f2)
}
```
The references `ts.f1` and `ts.f2` are implemented as method calls on the interface value `ts`. The methods are implemented as the obvious operations on the underlying type.
The only types that can be converted to `TS` are structs with the same field names and field types as `S` (the structs may have other fields as well).
Embedding multiple struct types in an interface type can only be implemented by a struct with all the listed fields. Embedding a struct and a map type, or other combinations, can not be implemented by any type, and is invalid.
For an embedded pointer type, an indirection on the pointer returns an interface value embedding the pointer target type. For example:
```Go
type S struct {
F int
}
type IP interface { *S }
type IS interface { S }
func F(ip IP) {
s := *ip // s has type IS
*ip = s // INVALID: s is type IS, but assignment requires type S
*ip = S{0} // OK
}
```
Values of one of these interface type may use a type assertion or type switch in the usual way to recover the original value.
This facility in itself is not particularly interesting (it does permit writing an interface type that implements "all structs with a field of name `F` and type `T`). To make it more interesting, we add the ability to embed only the getters of the various types, by using `const`.
```Go
type TS interface { const S }
```
Now the interface type provides only the methods that read fields of `S`, not the methods that change (or take the address of) fields. This then provides a way to pass a struct (or slice, etc.) to a function without giving the function the ability to change any elements.
Of course, the function can still use a type assertion or type switch to uncover the original value and modify fields that way. Or the function can use the reflect package similarly. So this is not a foolproof mechanism.
Nor should it be. For example, it can be useful to write `type ConstByteSlice interface { const []byte }` and to use that byte slice without changing it, while still preserving the ability to write `f.Write(cbs.([]byte))`, relying on the promise of the `Write` method without any explicit enforcement.
This ability to move back and forth permits adding "const-qualification" on a middle-out basis, without requiring it to be done entirely bottom-up. It also permits adding a mutation at the bottom of a stack of functions using a const interface, without requiring the whole stack to be adjusted, similar to C++ `const_cast`.
This is not immutability. If the value in the interface is a pointer or slice or map, the pointed-to elements may be changed by other aliases even if they are not changed by the const interface type.
This is, essentially, the ability to say "this function does not modify this aggregate value by accident (though it may modify it on purpose)." This is similar to the C/C++ `const` qualifier, but expressed as an interface type rather than as a type qualifier.
This is not generics or operator overloading.
One can imagine a number of other ways to adjust the methods attached to such an interface. For example, perhaps there would be a way to drop or replace methods selectively, or add advice to methods. We would have to work out the exact method names (required in any case for type reflection) and provide a way for people to write methods with the same names. That would come much closer to operator overloading, so it may or may not be a good idea. | LanguageChange,Proposal,LanguageChangeReview | high | Critical |
377,694,640 | node | discussion: put subsystem, team and label metadata in file(s) in this repo | Right now there are multiple places that somewhat capture the label and subsystem metadata in this repo:
In core-validate-commit:
https://github.com/nodejs/core-validate-commit/blob/4e64157b6a87267fd4b9f0598159eeb968e04e21/lib/rules/subsystem.js#L5-L68
In github-bot:
https://github.com/nodejs/github-bot/blob/8d4b7199f871fe37f2528520e79a0d8568960184/lib/node-labels.js#L5-L139
And of course the source of truth about labels is https://github.com/nodejs/node/labels
There is also discussion about automation of pinging teams, similar to how the now-removed CODEOWNERS file used to work (because the GitHub implementation didn't actually work for us). But to implement any of that, we will need this information actually written down somewhere in some format
https://github.com/nodejs/automation/issues/27
Also related: Store list of collaborators and TSC in a JSON file https://github.com/nodejs/node/issues/23623
Note that one of the reasons that CODEOWNERS is removed is that the pinging only worked for teams who have write access to this repo, and in core there are two type of groups we need to ping: WGs or teams whose members may or may not have write access but need to be informed (observers), and collaborators who tend to review the files more often than the others (owners).
At a glance, I think the information is basically a mapping of `file_pattern -> (subsystem, owners[], observers[], labels[])` - but maybe there is more e.g. precedence and hierarchy. (note that subsystems are not equivalent to labels, as labels can be `dont-land-*`)
I propose to put this information in this repo, and then we could figure out how to utilize it for automation - but it still has value even just as a general dictionary to look things up, even for humans.
On the automation's side - we could use it to ping people (or just suggest to you who to ping without actually pinging them so you don't accidentally create noise), to request reviews, to check reviews when landing PRs, to make sure the labels and their descriptions are not out of date, to dynamically introduce new subsystems in commit messages (to validate) etc. More importantly we can introduce new meta information and remove them by submitting PRs to these meta files instead of updating all the relevant projects (e.g. the bot doesn't know about all the files and the dont-land labels there are really out of date. If it just grabs the information from the master, we are more likely to keep the information up-to-date).
I don't know if there is a particularly good format, maybe a JSON or YAML? Or a variant of the removed CODEOWNERS (see https://github.com/nodejs/node/pull/21161) file?
For reference, WPT uses a [META.yml](https://github.com/web-platform-tests/wpt/blob/master/url/META.yml), v8 uses [OWNERS](https://github.com/v8/v8/blob/master/src/OWNERS), both seem to be home-grown files placed in different level of directories.
| discuss,meta | low | Major |
377,708,677 | vscode | [theme] inspect tool for workbench colors | Issue Type: Feature Request
Relevant Issues: There was one but I'm unable to find it, this is an idea to perhaps explore it. Maybe the bot can find it.
## Live Editor Theming
The original issue puts forward the idea of live editing a theme, such that when a change is applied, it's applied automatically without reloads and allows the developee to see what exactly changed. There are still a few downsides that I feel this feature request would solve. But before I explain, I'll describe my idea to push it further.
## Idea In-depth
My plan is to perhaps be able to have a 'Theme Dev Mode' of some sorts where the editor is put into a 'frozen' state (by this, I don't mean a 'Not Responding' state, but rather you can't type, UI actions don't do anything but are toggleable, basically a disabled state) and devs are able to click on various UI elements or scopes to open a pop up detailing all the possible relevant scopes that can be defined.
This is similar to the current `Developer: Inspect TM Scopes` but adds in support for UI too, and would be part of the 'live' experience
## How would this benefit developers
The original issue stated that it would help theme developers (the live theme idea in general), and I believe this notion. would. boost the efficiency further. Currently many devs probably go to the docs to find their relevant UI scope, but this could be made easier as the pop ups detailed here could also be powered by docs.
## Issues this specific idea would solve
(relating to this idea, not necessarily the original issue)
- Time consumed searching for scopes
- Much more friendly towards new devs
Cons:
- May be hard to implement, though the 'disabled' state is one thatvs started groundwork in the Walkthrough on the Welcome page | feature-request,themes | low | Major |
377,725,748 | godot | Some shortcuts on macOS don't work | **Godot version:**
3.0.6
**OS/device including version:**
mac os x, "High Sierra", Version 10.13.6 (17G2307)
**Issue description:**
I'm facing the issue that some shortcuts on my mac don't work though they are configured in the editor settings as shortcuts.
Examples of working and not working shortcuts::
ctrl+cmd+D - toggle fullscreen: works
ctrl+cmd+D - switch to distraction free view: does not work
shift+cmd+O - open scene: works
cmd+y - restore after undo: works
cmd+y - custom shortcut to delete line: does not work, though the default behaviour for cmd-y (restore) has been removed
There are also shortcuts that have an _unexpected_ behaviour. For example pressing alt+1 inside the text editor. Instead of switching to the 2d scene editor a special char is insert.
And I also would like to add a *comment* regarding cmd+left and cmd+right and cmd+backspace:
cmd+left/right works in the text editor, but (mostly) not in editor form fields.
cmd+backspace deletes on mac os the whole line. No effect in Godot (text editor included), although if I set cmd+backspace in the preferences to delete a line
**Steps to reproduce:**
Open the script editor and press the hotkeys
| bug,platform:macos,topic:editor,confirmed | medium | Major |
377,739,572 | TypeScript | Annoyingly useless Symbol completions | ```ts
""./**/
```
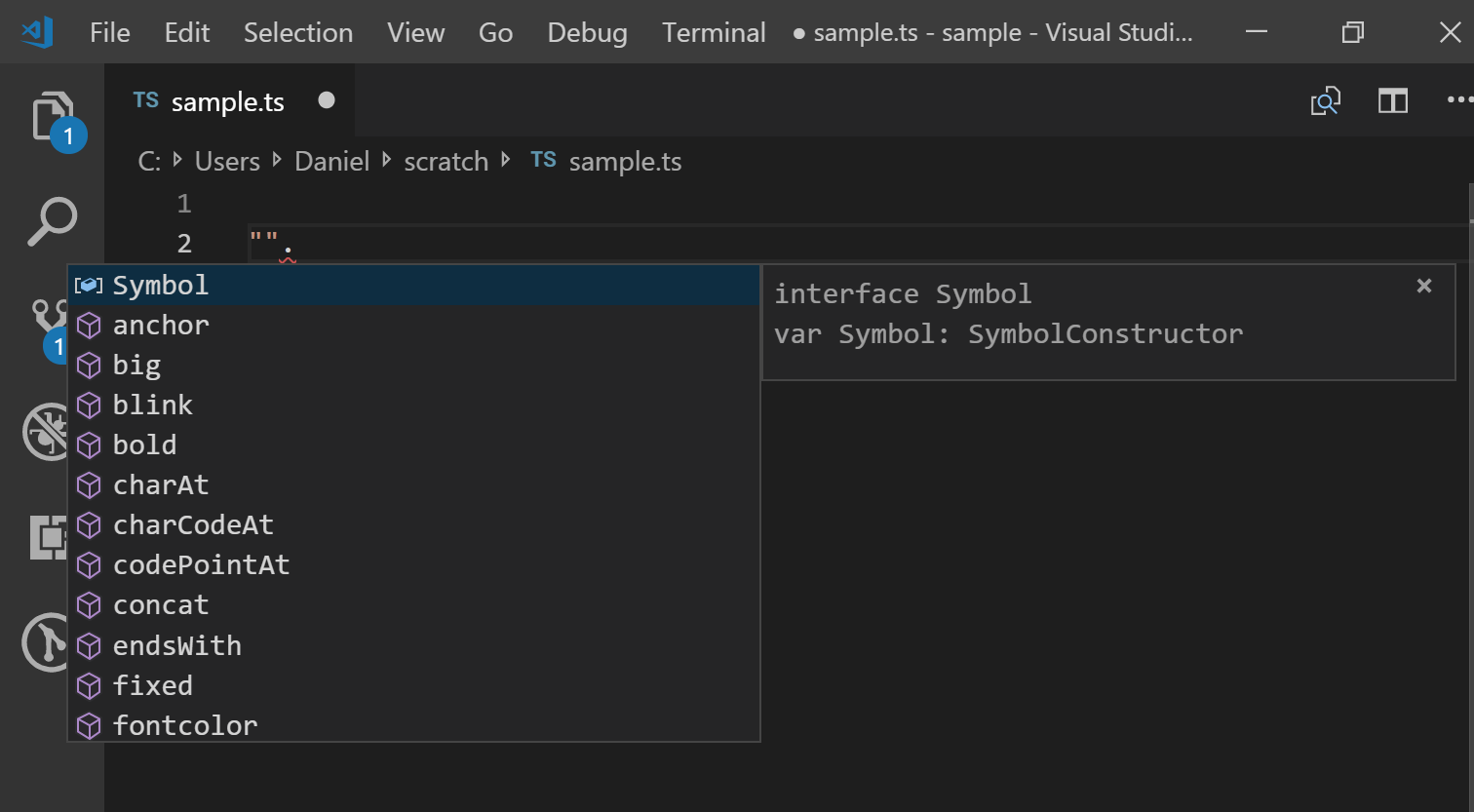
I guess the way I think about it, element access completions are pretty uncommon and should be considered lower-priority; and yet, they're the top item I'm given in a completion list. Is this for discoverability?
Additionally, if I actually commit to these completions, I end up *outside* of the place I want to continue typing:
```ts
""[Symbol]/*we currently end up here!*/
""[Symbol/*rather than here!*/]
""[Symbol./*or potentially here!*/]
``` | Bug,Help Wanted,Effort: Moderate,Domain: Completion Lists | low | Minor |
377,751,819 | TypeScript | API: Allow passing TypeScript.SourceFile / AST directly to transpileModule | <!-- π¨ STOP π¨ π¦π§π’π£ π¨ πΊπ»πΆπ· π¨
Half of all issues filed here are duplicates, answered in the FAQ, or not appropriate for the bug tracker. Please read the FAQ first, especially the "Common Feature Requests" section.
-->
## Search Terms
transpileModule directly AST SourceFile source performance
<!-- List of keywords you searched for before creating this issue. Write them down here so that others can find this suggestion more easily -->
## Suggestion
<!-- A summary of what you'd like to see added or changed -->
Currently, [`TypeScript.transpileModule`](https://github.com/Microsoft/TypeScript/blob/865b3e786277233585e1586edba52bf837b61b71/src/services/transpile.ts#L26) accepts string and option as parameters. It would be nice to have another method like this which can accept `TypeScript.SourceFile` directly.
## Use Cases
<!--
What do you want to use this for?
What shortcomings exist with current approaches?
-->
Currently I'm building [a parser which accepts JS](https://github.com/ryanelian/instapack/blob/terser/src/SyntaxLevelChecker.test.ts) for scanning whether ECMAScript syntax above user's project language level / `tsconfig.json:target` is used. *(For example: Your project targets ES2015 but one of the library imported uses ES2017 async-await syntax)*
When benchmarked, simply scanning the AST is faster (medium-sized project: 2s. no scan: 1 second) rather than straight-up transpiling every single library in node_modules (same project: 8s). Then, I only need to transpile JS files above project target level for optimal build performance!
It would be nice if I can pass the AST which I obtained from above process straight to `transpileModule` to prevent double parsing. (Especially since `transpileModule` uses some internal options such as `suppressOutputPathCheck` and `allowNonTsExtensions`)
## Examples
<!-- Show how this would be used and what the behavior would be -->
Proposed API:
```ts
transpileModuleAst(source: TypeScript.SourceFile, transpileOptions: TypeScript.transpileOptions): TypeScript.TranspileOutput
```
## Checklist
My suggestion meets these guidelines:
* [x] This wouldn't be a breaking change in existing TypeScript / JavaScript code
* [x] This wouldn't change the runtime behavior of existing JavaScript code
* [x] This could be implemented without emitting different JS based on the types of the expressions
* [x] This isn't a runtime feature (e.g. new expression-level syntax)
| Suggestion,In Discussion,API | low | Critical |
377,756,413 | flutter | flutter build apk / flutter install hangs on emulator created by flutter | ## Steps to Reproduce
1. Create an android emulator via flutter: flutter emulator --create (I have to edit the config slightly as per https://github.com/flutter/flutter/issues/23988)
2. Launch the emulator: flutter emulator --launch flutter_emulator
3. Create application: flutter create flutter_build_apk_test
4. Verify that stuff works:
$ cd flutter_build_apk_test/
$ flutter run
(the app launches and looks fine) (exiting with 'q')
5. $ flutter build apk
6. $ flutter install
now it just hangs at `Installing build/app/outputs/apk/app.apk`
If on the other hand I launch another emulator I have laying around:
```
$ time flutter build apk
Initializing gradle... 0.6s
Resolving dependencies... 1.1s
Gradle task 'assembleRelease'...
Gradle task 'assembleRelease'... Done 3.8s
Built build/app/outputs/apk/release/app-release.apk (4.7MB).
real 0m6.437s
user 0m5.053s
sys 0m0.685s
$ time flutter install
Initializing gradle... 0.6s
Resolving dependencies... 1.1s
Installing app.apk to Android SDK built for x86 64...
Installing build/app/outputs/apk/app.apk... 5.1s
Error: ADB exited with exit code 1
adb: failed to install [pathto]/flutter_build_apk_test/build/app/outputs/apk/app.apk: Failure [INSTALL_FAILED_NO_MATCHING_ABIS: Failed to
extract native libraries, res=-113]
Install failed
real 0m7.837s
user 0m3.766s
sys 0m0.742s
```
So here it just gives me a weird error --- but at least it doesn't hang.
The error is probably https://github.com/flutter/flutter/issues/8824
## Logs
With emulator that hangs:
```
$ flutter doctor -v
[β] Flutter (Channel master, v0.10.3-pre.104, on Linux, locale en_US.UTF-8)
β’ Flutter version 0.10.3-pre.104 at [pathto]/flutter/flutter
β’ Framework revision 7e652d1dba (8 hours ago), 2018-11-05 20:00:19 -0500
β’ Engine revision 3374f4cce0
β’ Dart version 2.1.0 (build 2.1.0-dev.8.0 bf26f760b1)
[β] Android toolchain - develop for Android devices (Android SDK 27.0.3)
β’ Android SDK at [pathto]/Android/Sdk
β’ Android NDK location not configured (optional; useful for native profiling support)
β’ Platform android-27, build-tools 27.0.3
β’ Java binary at: [pathto]/android-studio/jre/bin/java
β’ Java version OpenJDK Runtime Environment (build 1.8.0_112-release-b06)
β’ All Android licenses accepted.
[β] Android Studio (version 2.3)
β’ Android Studio at [pathto]/android-studio
β Flutter plugin not installed; this adds Flutter specific functionality.
β Dart plugin not installed; this adds Dart specific functionality.
β’ Java version OpenJDK Runtime Environment (build 1.8.0_112-release-b06)
[β] IntelliJ IDEA Ultimate Edition (version 2017.3)
β’ IntelliJ at /opt/intellij-ue-2017.3
β’ Flutter plugin version 20.0.2
β’ Dart plugin version 173.4700
[β] IntelliJ IDEA Ultimate Edition (version 2018.1)
β’ IntelliJ at /opt/intellij-ue-2018.1
β’ Flutter plugin version 29.0.2
β’ Dart plugin version 181.5540.11
[β] Connected device (1 available)
β’ Android SDK built for x86 β’ emulator-5554 β’ android-x86 β’ Android 8.1.0 (API 27) (emulator)
β’ No issues found!
```
With emulator that gives error:
```
$ flutter doctor -v
[β] Flutter (Channel master, v0.10.3-pre.104, on Linux, locale en_US.UTF-8)
β’ Flutter version 0.10.3-pre.104 at [pathto]/flutter/flutter
β’ Framework revision 7e652d1dba (8 hours ago), 2018-11-05 20:00:19 -0500
β’ Engine revision 3374f4cce0
β’ Dart version 2.1.0 (build 2.1.0-dev.8.0 bf26f760b1)
[β] Android toolchain - develop for Android devices (Android SDK 27.0.3)
β’ Android SDK at [pathto]/Android/Sdk
β’ Android NDK location not configured (optional; useful for native profiling support)
β’ Platform android-27, build-tools 27.0.3
β’ Java binary at: [pathto]/android-studio/jre/bin/java
β’ Java version OpenJDK Runtime Environment (build 1.8.0_112-release-b06)
β’ All Android licenses accepted.
[β] Android Studio (version 2.3)
β’ Android Studio at [pathto]/android-studio
β Flutter plugin not installed; this adds Flutter specific functionality.
β Dart plugin not installed; this adds Dart specific functionality.
β’ Java version OpenJDK Runtime Environment (build 1.8.0_112-release-b06)
[β] IntelliJ IDEA Ultimate Edition (version 2017.3)
β’ IntelliJ at /opt/intellij-ue-2017.3
β’ Flutter plugin version 20.0.2
β’ Dart plugin version 173.4700
[β] IntelliJ IDEA Ultimate Edition (version 2018.1)
β’ IntelliJ at /opt/intellij-ue-2018.1
β’ Flutter plugin version 29.0.2
β’ Dart plugin version 181.5540.11
[β] Connected device (1 available)
β’ Android SDK built for x86 64 β’ emulator-5554 β’ android-x64 β’ Android 7.1.1 (API 25) (emulator)
β’ No issues found!
``` | c: crash,platform-android,tool,P2,team-android,triaged-android | low | Critical |
377,777,480 | vscode | Regex replace in a large file clears an "innocent" line following a block of affected lines | Dear friends,
replacing a regex in a small file works fine, whereas doing so on a large file (originally 5.5M lines) results in incorrect behaviour 100% of time.
I have tried the steps below with the current VSCode release, the insiders build, and again the current release with extensions disabled, all on Win10 with the same (deterministic) result.
<!-- Please search existing issues to avoid creating duplicates. -->
<!-- Also please test using the latest insiders build to make sure your issue has not already been fixed: https://code.visualstudio.com/insiders/ -->
<!-- Use Help > Report Issue to prefill these. -->
Version: 1.28.2 (user setup)
- Commit: 7f3ce96ff4729c91352ae6def877e59c561f4850
- Date: 2018-10-17T00:23:51.859Z
- Electron: 2.0.9
- Chrome: 61.0.3163.100
- Node.js: 8.9.3
- V8: 6.1.534.41
- Architecture: x64
Version: 1.29.0-insider (user setup)
- Commit: bdfe4fd41f6a5fe538197b3cba937230249a563a
- Date: 2018-11-04T13:04:20.759Z
- Electron: 2.0.12
Steps to Reproduce:
1. Download, unzip, and open the [small file](https://github.com/Microsoft/vscode/files/2552357/odstavec_closing_tag_untouched.vert.txt) (29 lines) and the [large one](https://github.com/Microsoft/vscode/files/2552322/odstavec_closing_tag_cleared.zip) (6.3 M lines, 100 MiB), in which the content of the small file is simply duplicated more than 200,000 times. _The files contain vertical text: the first attested (Old) Czech sentence represented in tab-separated values wrapped in XML-like tags, a part of a text corpus._
2. Observe that on the small file, replacing the regex ^([^\t<]+).*$ with $1 works correctly. _The regex is supposed to strip annotation from non-XML lines (i.e. keep only the first column):_
**_a a|a|a k0|k8|k9_**
Sedlatu
.
</odstavec>
</folio>
becomes
**_a_**
Sedlatu
.
</odstavec>
</folio>
3. On the large file, not only the annotation is removed; lines with closing tags (</odstavec>) which immediately follow _affected_ lines are cleared as well:
**_a_**
Sedlatu
.
**[line cleared]**
</folio>
Thank you for resolving this issue!
PS: 7-Zip compresses the 100 MiB file to a 15 KiB archive, ZIP creates a 447 KiB archive. | bug,editor-find | low | Minor |
377,792,634 | TypeScript | Using createProgram outside node environment throws unhelpful exception message | When running `createProgram` from inside my angular app I'm thrown the 'ts.sys is undefined' error. This error is not helpful since I'm not given the reason for it. It took me a lot of time researching and eventually a question on SO (https://stackoverflow.com/questions/52969177/typescript-createprogram-throwing-ts-sys-is-undefined) to get the answer.
A more elaborate error message would've had been of great help.
**TypeScript Version:** 3.1.3
**Search Terms:** 'ts.sys is undefined'
**Code**
```ts
try {
createProgram(Utils.getFileNames(), {
lib: ['lib.es6.d.ts']
// target: ScriptTarget.ES5,
// module: ModuleKind.CommonJS
});
} catch (ex) {
debugger;
}
```
**Expected behavior:**
An exception message explaining I should run this code from node environment or pass my own compiler.
**Actual behavior:**
Throws 'ts.sys is undefined'
| Suggestion,In Discussion,API | low | Critical |
377,795,917 | go | cmd/go: go list -m -json output changes if module version supplied | <!-- Please answer these questions before submitting your issue. Thanks! -->
### What version of Go are you using (`go version`)?
<pre>
$ go version
go version go1.11.1 linux/amd64
</pre>
### Does this issue reproduce with the latest release?
Yes
### What operating system and processor architecture are you using (`go env`)?
<details><summary><code>go env</code> Output</summary><br><pre>
$ go env
GOARCH="amd64"
GOBIN="/home/myitcv/go-modules-by-example/.bin"
GOCACHE="/home/myitcv/.cache/go-build"
GOEXE=""
GOFLAGS=""
GOHOSTARCH="amd64"
GOHOSTOS="linux"
GOOS="linux"
GOPATH="/home/myitcv/gostuff"
GOPROXY=""
GORACE=""
GOROOT="/home/myitcv/gos"
GOTMPDIR=""
GOTOOLDIR="/home/myitcv/gos/pkg/tool/linux_amd64"
GCCGO="gccgo"
CC="gcc"
CXX="g++"
CGO_ENABLED="1"
GOMOD="/home/myitcv/go-modules-by-example/go.mod"
CGO_CFLAGS="-g -O2"
CGO_CPPFLAGS=""
CGO_CXXFLAGS="-g -O2"
CGO_FFLAGS="-g -O2"
CGO_LDFLAGS="-g -O2"
PKG_CONFIG="pkg-config"
GOGCCFLAGS="-fPIC -m64 -pthread -fmessage-length=0 -fdebug-prefix-map=/tmp/go-build283609322=/tmp/go-build -gno-record-gcc-switches"
</pre></details>
### What did you do?
```
$ git clone https://github.com/myitcvscratch/usenonmod /tmp/usenonmod
Cloning into '/tmp/usenonmod'...
$ cd /tmp/usenonmod
$ git checkout 8f021b7b44b6abe1c2cc18d87e72eb28dd1f6a33
HEAD is now at 8f021b7... Initial commit
$ go mod tidy
go: downloading github.com/myitcvscratch/nonmod v0.0.0-20181105194257-9516961eb10a
go: downloading golang.org/x/tools v0.0.0-20181105194243-ebdbadb46e45
$ go list -m all
github.com/myitcvscratch/usenonmod
github.com/myitcvscratch/nonmod v0.0.0-20181105194257-9516961eb10a
golang.org/x/tools v0.0.0-20181105194243-ebdbadb46e45
$ go list -m -json golang.org/x/tools
{
"Path": "golang.org/x/tools",
"Version": "v0.0.0-20181105194243-ebdbadb46e45",
"Time": "2018-11-05T19:42:43Z",
"Indirect": true,
"Dir": "/gopath/pkg/mod/golang.org/x/[email protected]",
"GoMod": "/gopath/pkg/mod/cache/download/golang.org/x/tools/@v/v0.0.0-20181105194243-ebdbadb46e45.mod"
}
$ go list -m -json golang.org/x/[email protected]
{
"Path": "golang.org/x/tools",
"Version": "v0.0.0-20181105194243-ebdbadb46e45",
"Time": "2018-11-05T19:42:43Z",
"Dir": "/gopath/pkg/mod/golang.org/x/[email protected]",
"GoMod": "/gopath/pkg/mod/cache/download/golang.org/x/tools/@v/v0.0.0-20181105194243-ebdbadb46e45.mod"
}
```
### What did you expect to see?
The same output for both `go list -m -json` commands.
### What did you see instead?
The `.Indirect` field is missing if the version is provided, as in the second call. | NeedsInvestigation,GoCommand,modules | low | Critical |
377,827,547 | electron | Too inconvenient to do conditional menu items using the menu template builder | **Is your feature request related to a problem? Please describe.**
I previously opened https://github.com/electron/electron/issues/13754, but instead of going with my preferred solution of filtering out falsy values, they went with strict type checking, which is fine, but it also means it's very inconvenient to do inline conditional menus.
This is not possible:
```js
electron.Menu.buildFromTemplate([
{
type: 'separator',
},
isLoggedIn ? {
label: 'Foo'
} : undefined
]);
```
You might be fooled into thinking you can just do this:
```js
electron.Menu.buildFromTemplate([
{
type: 'separator',
},
{
label: 'Foo',
visible: isLoggedIn
}
]);
```
But that will still show the separator, even when `visible: false`:
<img width="377" alt="screen shot 2018-11-06 at 19 32 48" src="https://user-images.githubusercontent.com/170270/48064578-c223cd80-e1fa-11e8-9d14-e49a8e4044a6.png">
**Describe the solution you'd like**
I'd like Electron to either:
1. Allow `undefined` and filter it out (deeply/recursively). That would be my preferred and most convenient solution.
2. Add a `{type: 'empty'}` that is filtered out (deeply/recursively).
**Describe alternatives you've considered**
Remove moot `{type: 'separator}` items. For example, when there's is a separator and then an `{visible: false}` item at the end, the separator should be filtered out as otherwise it would look weird.
**Additional context**
Cross-platform apps have a lot of conditional menu items. It should not be this hard to do it.
| enhancement :sparkles: | low | Major |
377,892,534 | vscode | API should support to create a folder | feature-request,api,file-io,workspace-edit | low | Major |
|
377,906,583 | flutter | Keyboard submit is off the screen on android Wear 2 device. | Problem is on an Android Wear app, mobile is fine.
There is no way to enter any input, as when the keyboard appears, submit is off the screen. Example...
```dart
return MaterialApp(
home: Scaffold(
body: new TextField(
keyboardType: TextInputType.multiline,
maxLines: null,
),
)
);
```
Screenshot at https://stackoverflow.com/questions/53161631/flutter-reposition-keyboard-down
## Logs
```
LEO DLXX β’ QEV7N17B22001003 β’ android-arm β’ Android 8.0.0 (API 26)
sdk gwear x86 β’ emulator-5554 β’ android-x86 β’ Android 8.0.0 (API 26) (emulator)
moo@moo2 ~/AndroidStudioProjects/flutter_gps $ flutter run --verbose
[ +27 ms] executing: [/home/moo/android/flutter/] git rev-parse --abbrev-ref --symbolic @{u}
[ +27 ms] Exit code 0 from: git rev-parse --abbrev-ref --symbolic @{u}
[ ] origin/beta
[ ] executing: [/home/moo/android/flutter/] git rev-parse --abbrev-ref HEAD
[ +4 ms] Exit code 0 from: git rev-parse --abbrev-ref HEAD
[ ] beta
[ ] executing: [/home/moo/android/flutter/] git ls-remote --get-url origin
[ +4 ms] Exit code 0 from: git ls-remote --get-url origin
[ ] https://github.com/flutter/flutter.git
[ ] executing: [/home/moo/android/flutter/] git log -n 1 --pretty=format:%H
[ +4 ms] Exit code 0 from: git log -n 1 --pretty=format:%H
[ ] f37c235c32fc15babe6dc7b7bc2ee4387e5ecf92
[ ] executing: [/home/moo/android/flutter/] git log -n 1 --pretty=format:%ar
[ +5 ms] Exit code 0 from: git log -n 1 --pretty=format:%ar
[ ] 6 weeks ago
[ ] executing: [/home/moo/android/flutter/] git describe --match v*.*.* --first-parent --long --tags
[ +5 ms] Exit code 0 from: git describe --match v*.*.* --first-parent --long --tags
[ ] v0.9.4-0-gf37c235
[ +61 ms] executing: /media/files/Android/Sdk/platform-tools/adb devices -l
[ +6 ms] Exit code 0 from: /media/files/Android/Sdk/platform-tools/adb devices -l
[ ] List of devices attached
QEV7N17B22001003 device usb:2-1.5 product:sawshark model:LEO_DLXX device:sawshark transport_id:15
emulator-5554 device product:sdk_gwear_x86 model:sdk_gwear_x86 device:generic_x86 transport_id:17
[ +9 ms] More than one device connected; please specify a device with the '-d <deviceId>' flag, or use '-d all' to act on all devices.
[ +5 ms] /media/files/Android/Sdk/platform-tools/adb -s QEV7N17B22001003 shell getprop
[ +102 ms] ro.hardware = sawshark
[ +1 ms] ro.build.characteristics = nosdcard,watch
[ +1 ms] /media/files/Android/Sdk/platform-tools/adb -s emulator-5554 shell getprop
[ +15 ms] ro.hardware = ranchu
[ +1 ms] LEO DLXX β’ QEV7N17B22001003 β’ android-arm β’ Android 8.0.0 (API 26)
[ ] sdk gwear x86 β’ emulator-5554 β’ android-x86 β’ Android 8.0.0 (API 26) (emulator)
[ +10 ms] "flutter run" took 172ms.
```
```
[β] Flutter (Channel beta, v0.9.4, on Linux, locale en_GB.UTF-8)
β’ Flutter version 0.9.4 at /home/moo/android/flutter
β’ Framework revision f37c235c32 (6 weeks ago), 2018-09-25 17:45:40 -0400
β’ Engine revision 74625aed32
β’ Dart version 2.1.0-dev.5.0.flutter-a2eb050044
[!] Android toolchain - develop for Android devices (Android SDK 27.0.3)
β’ Android SDK at /media/files/Android/Sdk
β’ Android NDK location not configured (optional; useful for native profiling support)
β’ Platform android-27, build-tools 27.0.3
β’ ANDROID_HOME = /media/files/Android/Sdk
β’ Java binary at: /home/moo/android-studio/jre/bin/java
β’ Java version OpenJDK Runtime Environment (build 1.8.0_152-release-1136-b06)
! Some Android licenses not accepted. To resolve this, run: flutter doctor --android-licenses
[β] Android Studio (version 2.2)
β’ Android Studio at /media/files/android-studio
β Flutter plugin not installed; this adds Flutter specific functionality.
β Dart plugin not installed; this adds Dart specific functionality.
β’ Java version OpenJDK Runtime Environment (build 1.8.0_76-release-b03)
[β] Android Studio (version 3.2)
β’ Android Studio at /home/moo/android-studio
β’ Flutter plugin version 30.0.1
β’ Dart plugin version 181.5656
β’ Java version OpenJDK Runtime Environment (build 1.8.0_152-release-1136-b06)
[β] Connected devices (2 available)
β’ LEO DLXX β’ QEV7N17B22001003 β’ android-arm β’ Android 8.0.0 (API 26)
β’ sdk gwear x86 β’ emulator-5554 β’ android-x86 β’ Android 8.0.0 (API 26) (emulator)
! Doctor found issues in 1 category.
```
| a: text input,e: device-specific,platform-android,framework,engine,f: material design,a: fidelity,a: layout,has reproducible steps,P3,team-design,triaged-design,found in release: 3.22 | low | Major |
377,909,268 | neovim | :terminal command to jump between prompts | I think a pretty nice feature of the terminal mode (`:terminal`) would be the possibility to jump between prompts, when being in normal mode in the terminal buffer.
E.g. my window looks like so:
```
+-------------------------------------------------------------------------+
|$ ls |
|decode.c encode.c executor.c gc.c typval.c typval_encode.h |
|decode.h encode.h executor.h gc.h typval_encode.c.h typval.h |
|$ β |
| |
|--TERMINAL-- |
+-------------------------------------------------------------------------+
```
and I am in normal mode and type e.g. `:PrevPrompt` and get:
```
+-------------------------------------------------------------------------+
|$ βs |
|decode.c encode.c executor.c gc.c typval.c typval_encode.h |
|decode.h encode.h executor.h gc.h typval_encode.c.h typval.h |
|$ |
| |
|--TERMINAL-- |
+-------------------------------------------------------------------------+
```
Then I type e.g. `:NextPrompt` and would be at first position again:
```
+-------------------------------------------------------------------------+
|$ ls |
|decode.c encode.c executor.c gc.c typval.c typval_encode.h |
|decode.h encode.h executor.h gc.h typval_encode.c.h typval.h |
|$ β |
| |
|--TERMINAL-- |
+-------------------------------------------------------------------------+
```
Of course some can search `$` and jump between prompts but not in a clean way since the output of terminal commands could contain the prompt string (or parts of it). | enhancement,terminal | medium | Major |
377,913,538 | TypeScript | NonNullable isn't narrowing down object values' types for optional properties | <!-- π¨ STOP π¨ π¦π§π’π£ π¨ πΊπ»πΆπ· π¨
Half of all issues filed here are duplicates, answered in the FAQ, or not appropriate for the bug tracker. Even if you think you've found a *bug*, please read the FAQ first, especially the Common "Bugs" That Aren't Bugs section!
Please help us by doing the following steps before logging an issue:
* Search: https://github.com/Microsoft/TypeScript/search?type=Issues
* Read the FAQ: https://github.com/Microsoft/TypeScript/wiki/FAQ
Please fill in the *entire* template below.
-->
<!-- Please try to reproduce the issue with `typescript@next`. It may have already been fixed. -->
**TypeScript Version:** 3.2.0-dev.20181106
<!-- Search terms you tried before logging this (so others can find this issue more easily) -->
**Search Terms:**
NonNullable object
NonNullable object values
**Code**
Run the following code via `tsc --no-emit --strict test.ts`:
```ts
interface P {
color?: 'red' | 'green';
}
type RequiredP = {
[K in keyof P]: NonNullable<P[K]>;
}
declare const p: RequiredP;
const color: 'red' | 'green' = p.color;
```
**Expected behavior:**
The resulting type should not allow `undefined` for the value at a property `color`.
**Actual behavior:**
`undefined` is still allowed. Using the `NonNullable` type doesn't seem to have any effect.
**Playground Link:** Note: you need to enable `strictNullChecks` manually!
https://www.typescriptlang.org/play/#src=interface%20P%20%7B%0D%0A%20%20color%3F%3A%20'red'%20%7C%20'green'%3B%0D%0A%7D%0D%0A%0D%0Atype%20RequiredP%20%3D%20%7B%0D%0A%20%20%5BK%20in%20keyof%20P%5D%3A%20NonNullable%3CP%5BK%5D%3E%3B%0D%0A%7D%0D%0A%0D%0Adeclare%20const%20p%3A%20RequiredP%3B%0D%0Aconst%20color%3A%20'red'%20%7C%20'green'%20%3D%20p.color%3B%0D%0A
**Related Issues:** <!-- Did you find other bugs that looked similar? -->
| Suggestion,Domain: Mapped Types,Experience Enhancement | medium | Critical |
377,924,286 | TypeScript | Introduce the opposite of the non-null assertion | ## Search Terms
non-null assertion opposite
## Suggestion
The opposite of the "non-null assertion", used to force the compiler to believe that something may be undefined.
The most obvious syntax would be a "?" postfix operator, similar to how the non-null assertion is a "!" postfix operator. This would be consistent with the use of "?" for optional function params and object properties (which adds "undefined" to the type).
## Use Cases
Indexing into arrays and "dictionary" objects with indexes/keys that are expected to potentially not exist.
Arrays and dictionary objects (an object type with the string index defined) are typically defined as having items whose type does NOT include undefined, even though it is technically possible (and valid) to use a non-existent index/key and get an undefined value as a result. In most situations, this avoids unnecessary/tedious non-null assertions, because arrays are often iterated (indexes guaranteed to exist), and dictionary objects are often used only to lookup keys that are guaranteed to exist.
However, in situations where an "untrusted" index/key is used on an array/dictionary, there is no convenient way to alter the type of the expression to indicate that the programmer is aware that the result may be undefined:
## Examples
```ts
declare const array: Date[];
declare const untrustedIndex: number;
// Type is Date, but it may actually be undefined at runtime.
const item = array[untrustedIndex];
// To get useful "correct" typing, I must explicitly cast, duplicating the type
// of the item and adding "| undefined"
const item2 = array[untrustedIndex] as Date | undefined;
// Using the new proposed "possibly undefined assertion"
// Type is Date | undefined
const item3 = array[untrustedIndex]?;
```
## Checklist
My suggestion meets these guidelines:
* [?] This wouldn't be a breaking change in existing TypeScript / JavaScript code
* [x] This wouldn't change the runtime behavior of existing JavaScript code
* [x] This could be implemented without emitting different JS based on the types of the expressions
* [x] This isn't a runtime feature (e.g. new expression-level syntax)
| Suggestion,Awaiting More Feedback | low | Minor |
377,935,770 | vue | vnode reference to original component (reopened) | ### What problem does this feature solve?
Because we have to have lots of ads on our site we cannot hydrate our SSR'd page with VUE completely as the ads would break VUE. Also most of our site ist static and it would be stupid to ship our entire app for only some parts of our page being interactive.
Instead we **partially hydrate** the page (with our own plugin https://github.com/spring-media/vue-plugin-moisturizer). For this we need to map SSR'd HTML to the corresponding VUE components, so we need to put some `data-attribute` in the HTML match them.
We use the `name` setting of a component for this, so if I have component like this:
```js
{
name: 'my-component'
}
```
the html would read
```html
<div data-hydration-name="my-component">...</div>
```
but this does not work for 3rd party components. Anything we get without that ID we cannot hydrate. It would be great if we could find the component from a component's instance / vnode.
There is a `cid` property, but this is not the same on the server and on the client, because we have a different number and oder of components on the client and server so we cannot use this.
There is a similiar issue for this here: https://github.com/vuejs/vue/issues/7213 but one of your members suggested to create a new issue because the old one would just not show up under your pile of issues.
### What does the proposed API look like?
```js
this.$vnode.cuuid // hash based on the filename and path
```
<!-- generated by vue-issues. DO NOT REMOVE --> | feature request | low | Minor |
377,949,732 | TypeScript | infer-from-usage codefix should mark parameters as optional | ## Search Terms
codefix infer from usage optional parameter
## Suggestion
When you ask to for the infer-from-usage codefix for `f`:
```ts
function f(x, y) {
return x + (y || 0)
}
f(1)
```
You should get `function f(x: number, y?: number | undefined)`
This already works in JS. You get `/** @param {number | undefined} [y] */`. | Suggestion,In Discussion,Domain: Quick Fixes | low | Minor |
377,956,201 | rust | Tracking issue for alloc_layout_extra | This issue tracks additional methods on `Layout` which allow layouts to be composed to build complex layouts.
```rust
pub const fn padding_needed_for(&self, align: usize) -> usize;
pub const fn repeat(&self, n: usize) -> Result<(Layout, usize), LayoutErr>;
pub const fn repeat_packed(&self, n: usize) -> Result<Layout, LayoutErr>;
pub const fn extend_packed(&self, next: Layout) -> Result<Layout, LayoutErr>;
pub const fn dangling(&self) -> NonNull<u8>;
```
The main use case is to construct complex allocation layouts for use with the stable global allocator API. For example:
- [`std::collections::HashMap`](https://github.com/rust-lang/rust/blob/master/src/libstd/collections/hash/table.rs#L657)
- [`hashbrown`](https://github.com/Amanieu/hashbrown/blob/2f2af1d1e9bea9d7315b5a85d9cf5b5514f532fe/src/raw/mod.rs#L143)
- Not exactly an example of use, but it would be very useful in [`crossbeam-skiplist`](https://github.com/crossbeam-rs/crossbeam-skiplist/blob/master/src/base.rs#L99)
One concern is that not many of these methods have been extensively used in practice. In the examples given above, only `extend`, `array` and `align_to` are used, and I expect that these will be the most used in practice. `padding_needed_for` is used in the implementation of `Rc::from_raw` and `Arc::from_raw`, but in theory could be superseded by the offset returned by `extend`. | A-allocators,T-libs-api,B-unstable,C-tracking-issue,Libs-Tracked | medium | Critical |
377,987,649 | react | Autofocus Text puts cursor at end instead of beginning | **Do you want to request a *feature* or report a *bug*?**
Bug
**What is the current behavior?**
In React 16, a text input with a value and autofocus will set the cursor at the _end_ of the input.
`<input autoFocus={true} type="text" defaultValue="4444" />`
**If the current behavior is a bug, please provide the steps to reproduce and if possible a minimal demo of the problem. Your bug will get fixed much faster if we can run your code and it doesn't have dependencies other than React. Paste the link to your JSFiddle (https://jsfiddle.net/Luktwrdm/) or CodeSandbox (https://codesandbox.io/s/new) example below:**
See the React 16 repro here: https://codepen.io/matthewg0/pen/XymjYo
**What is the expected behavior?**
In React 15, and in plain HTML, the behavior is that the cursor is placed at the _start_ of the input.
React 15: https://codepen.io/matthewg0/pen/NEGRzv
Plain HTML: https://codepen.io/matthewg0/pen/wQKoap
**Which versions of React, and which browser / OS are affected by this issue? Did this work in previous versions of React?**
Reproduces as of React 16. Worked as expected in React 15. | Component: DOM,Type: Regression | low | Critical |
378,004,413 | go | proposal: runtime: provide access to info about recent GC cycles | Currently, `runtime.parsedebugvars` is called from `schedinit` which is part of binary bootstrap. This means it's not possible to dynamically enable GODEBUG features once a binary has started.
Typically, we don't want gc traces to flood our logs, but when debugging specific memory issues, it would be helpful if we could enable features (such as gctrace=1 and scavenge=1) dynamically.
While it doesn't have to be via `os.Setenv`, it;d be useful to have some way to dynamically enable `GODEBUG` features.
### What version of Go are you using (`go version`)?
```
$ go version
go version go1.11.2 linux/amd64
```
### Does this issue reproduce with the latest release?
Yes, I'm using the latest release.
### What operating system and processor architecture are you using (`go env`)?
```
$ go env
GOARCH="amd64"
GOBIN=""
GOCACHE="/home/prashant/.cache/go-build"
GOEXE=""
GOFLAGS=""
GOHOSTARCH="amd64"
GOHOSTOS="linux"
GOOS="linux"
GOPATH="/home/prashant/go"
GOPROXY=""
GORACE=""
GOROOT="/home/prashant/bin/go1.11.2"
GOTMPDIR=""
GOTOOLDIR="/home/prashant/bin/go1.11.2/pkg/tool/linux_amd64"
GCCGO="gccgo"
CC="gcc"
CXX="g++"
CGO_ENABLED="1"
GOMOD=""
CGO_CFLAGS="-g -O2"
CGO_CPPFLAGS=""
CGO_CXXFLAGS="-g -O2"
CGO_FFLAGS="-g -O2"
CGO_LDFLAGS="-g -O2"
PKG_CONFIG="pkg-config"
GOGCCFLAGS="-fPIC -m64 -pthread -fmessage-length=0 -fdebug-prefix-map=/tmp/go-build849457703=/tmp/go-build -gno-record-gcc-switches"
```
### What did you do?
Set `GODEBUG` to `gctrace=1,scavenge=1` dynamically using `os.Setenv`.
### What did you expect to see?
Expected to see GC traces once the variable was set.
### What did you see instead?
No GC traces. | Proposal,Proposal-Hold | low | Critical |
378,023,138 | go | fmt: compound object rules don't seem to apply recursively | ERROR: type should be string, got "https://golang.org/pkg/fmt/#hdr-Printing reads:\r\n\r\n> For compound objects, the elements are printed using these rules, recursively, laid out like this:\r\n> ```\r\n> struct: {field0 field1 ...}\r\n> array, slice: [elem0 elem1 ...]\r\n> maps: map[key1:value1 key2:value2 ...]\r\n> pointer to above: &{}, &[], &map[]\r\n> ```\r\n\r\nIt is specifically said that these rules apply recursively. However, take a look at this playground link: https://play.golang.org/p/CbrniH9q45I\r\n\r\nIt currently prints:\r\n\r\n```\r\n{x}\r\n&{x}\r\n{%!s(*main.T2=&{x})}\r\n&{%!s(*main.T2=&{x})}\r\n```\r\n\r\nBut I'd expect it to print:\r\n\r\n```\r\n{x}\r\n&{x}\r\n{&{x}}\r\n&{&{x}}\r\n```\r\n\r\nReading the docs carefully again, I can't find a reason why only top-level pointers would follow the \"pointer to above\" rule that's clearly documented.\r\n\r\nIt seems to me like either the code is wrong, or the docs need clarification. If only top-level pointers are supposed to follow the \"pointer to above\" rule, that should be made clear in the rules.\r\n\r\nThis issue is split from #27672. I initially thought this was a bug in vet, but then started wondering if this was a bug in fmt instead.\r\n\r\n/cc @robpike @martisch @rogpeppe " | Documentation,NeedsFix | low | Critical |
378,023,213 | TypeScript | "Unique symbol as the name of a private method" results in invalid .d.ts declaration | <!-- Please try to reproduce the issue with `typescript@next`. It may have already been fixed. -->
**TypeScript Version:** 3.1.6 or 3.2.0-dev.20181106
<!-- Search terms you tried before logging this (so others can find this issue more easily) -->
**Search Terms:**
- unique symbol
- private class method name
- private symbol bad export
- bad code generation
## Use case
Using a `unique symbol` as the name of a private class method (on an exported class)
## Issue
The private method is _incorrectly_ exposed in the `.d.ts` module; as in, the unique symbol is used in the `.d.ts` module but never declared.
This means the exported class cannot be used by other TypeScript packages without a compiler error.
### [_Link to repro_](https://github.com/aleclarson/repro/tree/ts-issue-28383)
## Related Issues
*Unknown* | Bug,Domain: Declaration Emit | low | Critical |
378,040,402 | go | cmd/compile: liveness analysis conservative for compound objects | ```
func f(data []byte) {
dataSize := len(data)
g(data)
runtime.GC()
global = dataSize
}
func g([]byte)
var global int
```
The compiler reports (with `-live`) that `data` is live at the `runtime.GC` call. In fact, only the `.len` field of `data` is live at that point. But because we track liveness by variable, if any field of that variable is live whole variable is live. This means `data` will be retained unnecessarily by the garbage collector.
The generated code contains:
```
0x001d 00029 (tmp1.go:7) MOVQ "".data+40(SP), AX
0x0022 00034 (tmp1.go:7) MOVQ AX, (SP)
0x0026 00038 (tmp1.go:7) MOVQ "".data+48(SP), AX
0x002b 00043 (tmp1.go:7) MOVQ AX, 8(SP)
0x0030 00048 (tmp1.go:7) MOVQ "".data+56(SP), CX
0x0035 00053 (tmp1.go:7) MOVQ CX, 16(SP)
0x003a 00058 (tmp1.go:7) CALL "".g(SB)
0x003f 00063 (tmp1.go:8) CALL runtime.GC(SB)
0x0044 00068 (tmp1.go:9) MOVQ "".data+48(SP), AX
0x0049 00073 (tmp1.go:9) MOVQ AX, "".global(SB)
```
Even though the code looks like it reads the length first, the compiler postpones that read until after the `runtime.GC` call (so it doesn't have to issue a pointless spill).
Two solutions come to mind. One is to track liveness of individual pointer fields of variables, instead of whole variables. That would work, but could be expensive for variables which have lots of pointer fields. It also isn't possible to do precisely for variables which are arrays of pointers and have non-constant indexes.
The other solution is to ignore reads of scalars when doing the liveness analysis, as the results of the liveness analysis are only used by the GC. This is more of a hack but would be a simpler fix for the code in this issue.
| GarbageCollector,compiler/runtime | low | Major |
378,069,219 | react-native | Fix StrictMode warnings | <!--
- [x] Review the documentation: https://facebook.github.io/react-native
- [x] Search for existing issues: https://github.com/facebook/react-native/issues
- [x] Use the latest React Native release: https://github.com/facebook/react-native/releases
-->
## Description
When wrapping the app with `<StrictMode>`, there are warnings coming from internal react native components. They need to be updated to stop using the [legacy context api](https://github.com/facebook/react-native/search?q=childContextTypes&unscoped_q=childContextTypes) and unsafe methods like [UNSAFE_componentWillReceiveProps](https://github.com/facebook/react-native/search?q=UNSAFE_componentWillReceiveProps&unscoped_q=UNSAFE_componentWillReceiveProps).
Also, I believe this is a prerequisite for Concurrent Mode support.
<div>
<img width="300" src="https://user-images.githubusercontent.com/619186/48099194-f486f800-e205-11e8-97da-a45ef2f25cb4.png" />
<img width="300" src="https://user-images.githubusercontent.com/619186/48099196-f486f800-e205-11e8-9277-d3cc15d1b9b4.png" />
</div>
<details>
<summary>Environment</summary>
## Environment
React Native Environment Info:
System:
OS: macOS 10.14
CPU: x64 Intel(R) Core(TM) i5-6267U CPU @ 2.90GHz
Memory: 33.30 MB / 16.00 GB
Shell: 3.2.57 - /bin/bash
Binaries:
Node: 8.11.4 - /usr/local/bin/node
Yarn: 1.10.1 - ~/.yarn/bin/yarn
npm: 6.4.1 - /usr/local/bin/npm
Watchman: 4.9.0 - /usr/local/bin/watchman
SDKs:
iOS SDK:
Platforms: iOS 12.1, macOS 10.14, tvOS 12.1, watchOS 5.1
Android SDK:
Build Tools: 23.0.1, 23.0.3, 24.0.3, 25.0.0, 25.0.1, 25.0.2, 25.0.3, 26.0.0, 26.0.1, 26.0.2, 26.0.3, 27.0.0, 27.0.1, 27.0.2, 27.0.3, 28.0.0, 28.0.2, 28.0.3
API Levels: 19, 22, 23, 24, 25, 26, 27, 28
IDEs:
Android Studio: 3.2 AI-181.5540.7.32.5014246
Xcode: 10.1/10B61 - /usr/bin/xcodebuild
npmGlobalPackages:
react-native-cli: 2.0.1
</details>
| Help Wanted :octocat:,Resolution: PR Submitted,Bug | medium | Major |
378,075,001 | go | fmt: differing output of printf %#010x with int and string types | #### What did you do?
I wanted print a padded (zeros) and prepended (0x) string character as a hex number.
[Link to example on play.golang.org](https://play.golang.org/p/RMzu8R3Q11M)
#### What did you expect to see?
The same result for a string character and an integer.
The string printed as an hexadecimal number should be prepended with '0x'.
#### What did you see instead?
```
> A hex Number (a int) with padding: 0x0000000030
> A hex Number (a string) with padding: 0000000x30
>
> Program exited.
```
#### System details
```
go version go1.11.2 linux/amd64
GOARCH="amd64"
GOBIN=""
GOCACHE="/home/sebi/.cache/go-build"
GOEXE=""
GOFLAGS=""
GOHOSTARCH="amd64"
GOHOSTOS="linux"
GOOS="linux"
GOPATH="/home/sebi/go/"
GOPROXY=""
GORACE=""
GOROOT="/usr/local/go"
GOTMPDIR=""
GOTOOLDIR="/usr/local/go/pkg/tool/linux_amd64"
GCCGO="gccgo"
CC="gcc"
CXX="g++"
CGO_ENABLED="1"
GOMOD=""
GOROOT/bin/go version: go version go1.11.2 linux/amd64
GOROOT/bin/go tool compile -V: compile version go1.11.2
uname -sr: Linux 4.15.0-38-generic
Distributor ID: Ubuntu
Description: Ubuntu 16.04.5 LTS
Release: 16.04
Codename: xenial
/lib/x86_64-linux-gnu/libc.so.6: GNU C Library (Ubuntu GLIBC 2.23-0ubuntu10) stable release version 2.23, by Roland McGrath et al.
gdb --version: GNU gdb (Ubuntu 7.11.1-0ubuntu1~16.5) 7.11.1
```
| Documentation,NeedsInvestigation | low | Major |
378,076,766 | electron | Allow data URLs or Object URLs to work with nodeIntegrationInWOrker | If you try to make a `new Worker` with, for example, an Object URL or Data URL instead of a more normal URL like a `file://` URL, then module resolution will not work inside the Worker.
**Example**
The following works:
```js
const path = require('path')
new Worker(path.resolve(__dirname, 'worker.js'))
```
The following should work (doesn't):
```js
const fs = require('fs')
const source = fs.readFileSync(path.resolve(__dirname, 'worker.js'), 'utf8')
const sourceUrl = URL.createObjectURL(
new Blob([source], { type: 'text/javascript' }),
)
const worker = new Worker(sourceUrl)
```
Here's a reproduction: https://github.com/trusktr/electron-web-worker-example/tree/electron-issue-15609.
To run it:
```sh
git checkout electron-issue-15609 # use this branch
npm install
npm start
```
then look at the console and you will see
```
Uncaught Error: Cannot find module 'lodash'
```
It doesn't work because the call to `Module._resolveLookupPaths` during module load is not able to find any `node_modules` folders. I suppose the object URL isn't relative to anywhere on the filesystem, so it has no way to know where to find `node_modules`.
How can we fix this? | enhancement :sparkles: | low | Critical |
378,078,871 | go | html/template: document that it strips comments | <!-- Please answer these questions before submitting your issue. Thanks! -->
### What version of Go are you using (`go version`)?
go1.11.1 (playground)
### Does this issue reproduce with the latest release?
Yes.
### What did you do?
```go
package main
import (
"fmt"
"html/template"
"os"
)
const templateSrc = `<style>/* one */</style> /* two */ <!-- three -->`
func main() {
fmt.Println(templateSrc)
tmpl := template.Must(template.New("").Parse(templateSrc))
fmt.Println(tmpl.Tree.Root.String())
tmpl.Execute(os.Stdout, nil)
}
```
https://play.golang.org/p/WPnEwLYTBkF
### What did you expect to see?
Comments preserved in output.
```text
<style>/* one */</style> /* two */ <!-- three -->
<style>/* one */</style> /* two */ <!-- three -->
<style>/* one */</style> /* two */ <!-- three -->
```
### What did you see instead?
Comments are stripped.
```text
<style>/* one */</style> /* two */ <!-- three -->
<style>/* one */</style> /* two */ <!-- three -->
<style> </style> /* two */
```
---
This behaviour is apparently intentional (https://github.com/golang/go/issues/14256), but it is surprising and undocumented. Templates already have their own special comment syntax (`{{/* */}}`), so i didn't expect that they would gobble up HTML and CSS (and presumably JS) comments as well. This needs to be called out in the documentation, ideally with a rationale and workaround. | Documentation,help wanted,NeedsFix | low | Major |
378,111,625 | pytorch | [caffe2] How to export onnx model trained on Detectron in Caffe2? | caffe2 | low | Minor |
|
378,112,287 | rust | Distributed libLLVM conflicts with system libraries | Now that `libLLVM.so` is distributed with rust, we are unable to dynamically link a rust binary against a different `libLLVM.so` library. The rustlib directory containing `libLLVM` is prepended to the linker search path, and I don't see a good way to ask cargo to add a search path earlier than the rustlib is added. It looks to me like the rustlib directory is added here: https://github.com/rust-lang/rust/blob/15d770400eed9018f18bddf83dd65cb7789280a5/src/librustc_codegen_llvm/back/link.rs#L1044
I noticed that support for appending a suffix to the LLVM libs was added in https://github.com/rust-lang/rust/pull/53987. Can we turn that on for CI so the prebuilt distributions have a suffix on the library?
Unfortunately, the suffix won't actually fix this issue on MacOS, since the LLVM build does not append the suffix (or any version info) to the name of the dynamic library when building on MacOS: (see https://github.com/rust-lang/llvm/blob/7051ead40a5f825878b59bf08d4e768be9e99a4a/cmake/modules/AddLLVM.cmake#L520) | A-linkage,A-LLVM,T-compiler | low | Major |
378,120,871 | go | cmd/compile: thread support for webassembly | <!-- Please answer these questions before submitting your issue. Thanks! -->
### What version of Go are you using (`go version`)?
<pre>
$ go version
go version go1.11.1 darwin/amd64
</pre>
### Does this issue reproduce with the latest release?
Yes
### What operating system and processor architecture are you using (`go env`)?
<details><summary><code>go env</code> Output</summary><br><pre>
$ go env
GOARCH="amd64"
GOBIN=""
GOCACHE="/Users/sion/Library/Caches/go-build"
GOEXE=""
GOFLAGS=""
GOHOSTARCH="amd64"
GOHOSTOS="darwin"
GOOS="darwin"
GOPATH="/Users/sion/go1.X"
GOPROXY=""
GORACE=""
GOROOT="/usr/local/go"
GOTMPDIR=""
GOTOOLDIR="/usr/local/go/pkg/tool/darwin_amd64"
GCCGO="gccgo"
CC="clang"
CXX="clang++"
CGO_ENABLED="1"
GOMOD=""
CGO_CFLAGS="-g -O2"
CGO_CPPFLAGS=""
CGO_CXXFLAGS="-g -O2"
CGO_FFLAGS="-g -O2"
CGO_LDFLAGS="-g -O2"
PKG_CONFIG="pkg-config"
GOGCCFLAGS="-fPIC -m64 -pthread -fno-caret-diagnostics -Qunused-arguments -fmessage-length=0 -fdebug-prefix-map=/var/folders/6z/bfzgs4bs75bdjmr9c7dggct40000gn/T/go-build090156779=/tmp/go-build -gno-record-gcc-switches -fno-common"
</pre></details>
### What did you do?
It is very nice to use goroutine browsers, [WebAssembly Threads ready to try in Chrome 70](https://developers.google.com/web/updates/2018/10/wasm-threads). I found no related issues here, so i just add this issue.
<!--
If possible, provide a recipe for reproducing the error.
A complete runnable program is good.
A link on play.golang.org is best.
-->
### What did you expect to see?
Thread feature supported in xx.wasm.
### What did you see instead?
Not supported in chromium 72.
| NeedsDecision,FeatureRequest,arch-wasm,compiler/runtime | medium | Critical |
378,149,115 | rust | Providing the compiler crates as rlib? | I'd like to embed `rustc_driver` in a static library (for complicated embedded deployment reasons that aren't entirely relevant). Dependencies of a static library seem to have to be in rlib format so the compile can read metadata.
Unfortunately, most of the compiler crates are only building dylibs, causing cargo to refuse to build a staticlib:
```
error: crate `rustc_driver` required to be available in rlib format, but was not found in this form
error: crate `arena` required to be available in rlib format, but was not found in this form
error: crate `rustc_data_structures` required to be available in rlib format, but was not found in this form
error: crate `graphviz` required to be available in rlib format, but was not found in this form
error: crate `rustc_cratesio_shim` required to be available in rlib format, but was not found in this for
error: crate `rustc` required to be available in rlib format, but was not found in this form
error: crate `fmt_macros` required to be available in rlib format, but was not found in this form
error: crate `rustc_target` required to be available in rlib format, but was not found in this form
error: crate `rustc_errors` required to be available in rlib format, but was not found in this form
error: crate `syntax_pos` required to be available in rlib format, but was not found in this form
error: crate `syntax` required to be available in rlib format, but was not found in this form
error: crate `proc_macro` required to be available in rlib format, but was not found in this form
error: crate `rustc_fs_util` required to be available in rlib format, but was not found in this form
error: crate `rustc_allocator` required to be available in rlib format, but was not found in this form
error: crate `rustc_borrowck` required to be available in rlib format, but was not found in this form
error: crate `rustc_mir` required to be available in rlib format, but was not found in this form
error: crate `rustc_passes` required to be available in rlib format, but was not found in this form
error: crate `rustc_lint` required to be available in rlib format, but was not found in this form
error: crate `rustc_plugin` required to be available in rlib format, but was not found in this form
error: crate `rustc_metadata` required to be available in rlib format, but was not found in this form
error: crate `syntax_ext` required to be available in rlib format, but was not found in this form
error: crate `rustc_privacy` required to be available in rlib format, but was not found in this form
error: crate `rustc_typeck` required to be available in rlib format, but was not found in this form
error: crate `rustc_platform_intrinsics` required to be available in rlib format, but was not found in this form
error: crate `rustc_incremental` required to be available in rlib format, but was not found in this form
error: crate `rustc_resolve` required to be available in rlib format, but was not found in this form
error: crate `rustc_save_analysis` required to be available in rlib format, but was not found in this form
error: crate `rustc_codegen_utils` required to be available in rlib format, but was not found in this form
error: crate `rustc_traits` required to be available in rlib format, but was not found in this form
error: aborting due to 29 previous errors
```
Is there a technical reason why these are all only built as dylib, or is this just because no one has thus far needed to embed them in a staticlib? Would it be a reasonable ask to configure these projects to build both flavours? | T-compiler,T-bootstrap,C-feature-request | low | Critical |
378,173,844 | rust | There's no good way to iterate over all newlines with BufRead | If you have files with a newline of '\r', the only way to handle them is to use split. But if you have files with a newline of '\n' or '\r\n', then you can use lines().
This is counterintuitive and problematic from the perspective of the BufRead interface - there's no way to use it to iterate over any of the three types. I'd typically expect that lines() would handle this transparently.
I'm not sure if this is the right place, but I don't want it to get lost because it cost me major hassle. | T-libs-api,A-str,A-io | low | Major |
378,218,629 | flutter | Use GestureDetector and CustomScrollView together | Hello,
I try use customScrollView for pull-to-refreshοΌsee code
```
CustomScrollView(
shrinkWrap: widget.shrinkWrap,
physics: RefreshScrollPhysics(),
slivers: <Widget>[
CupertinoSliverRefreshControl(
builder: _buildSimpleRefreshIndicator,
onRefresh: () => Future(() => widget.loadNew())),
SliverSafeArea(sliver: SliverList(
delegate: SliverChildBuilderDelegate((context, index) {
return _buildProgressIndicator();
},
childCount: widget.itemCount)
))
],
controller: _scrollController,
)
```
But it drop down to a certain distance will refreshοΌWhat I want is to loosen my fingers and refreshγ And the refreshIndicatorMode has only five statesοΌThere is no one state indicating that the finger is off the screenγSo finallyοΌI use gestureDetector and customScrollView together to get the state of the fingerγ
```
GestureDetector(
child: CustomScrollView(...),
onVerticalDragDown: (_) {
print("show onVerticalDragDown");
},
onVerticalDragStart: (_) {
print("show onVerticalDragStart");
},
onVerticalDragUpdate: (_) {
print("show onVerticalDragUpdate");
},
onVerticalDragEnd: (_) {
print("show onVerticalDragEnd");
},
onVerticalDragCancel: () {
print("show onVerticalDragCancel");
},
)
```
But there seems to be a sliding conflictοΌitβs only print 'show onVerticalDragDown' and then print 'show onVerticalDragCancel'γSo how to solve these problems when can't use it to set physics is NeverScrollableScrollPhysicsγ
flutter doctor -v
```
[β] Flutter (Channel dev, v0.10.1, on Mac OS X 10.14 18A391, locale en-CN)
β’ Flutter version 0.10.1 at /Users/dpuntu/flutter
β’ Framework revision 6a3ff018b1 (3 weeks ago), 2018-10-18 18:38:26 -0400
β’ Engine revision 3860a43379
β’ Dart version 2.1.0-dev.7.1.flutter-b99bcfd309
[β] Android toolchain - develop for Android devices (Android SDK 28.0.3)
β’ Android SDK at /Users/dpuntu/Library/Android/sdk
β’ Android NDK location not configured (optional; useful for native profiling support)
β’ Platform android-28, build-tools 28.0.3
β’ ANDROID_HOME = /Users/dpuntu/Library/Android/sdk
β’ Java binary at: /Applications/Android Studio.app/Contents/jre/jdk/Contents/Home/bin/java
β’ Java version OpenJDK Runtime Environment (build 1.8.0_152-release-1024-b01)
β’ All Android licenses accepted.
[β] iOS toolchain - develop for iOS devices (Xcode 10.1)
β’ Xcode at /Applications/Xcode.app/Contents/Developer
β’ Xcode 10.1, Build version 10B61
β’ ios-deploy 1.9.2
β’ CocoaPods version 1.5.3
[β] Android Studio (version 3.1)
β’ Android Studio at /Applications/Android Studio.app/Contents
β’ Flutter plugin version 28.0.1
β’ Dart plugin version 173.4700
β’ Java version OpenJDK Runtime Environment (build 1.8.0_152-release-1024-b01)
[!] IntelliJ IDEA Community Edition (version 2017.3)
β’ IntelliJ at /Applications/IntelliJ IDEA CE.app
β Flutter plugin not installed; this adds Flutter specific functionality.
β Dart plugin not installed; this adds Dart specific functionality.
β’ For information about installing plugins, see
https://flutter.io/intellij-setup/#installing-the-plugins
[β] VS Code (version 1.28.2)
β’ VS Code at /Applications/Visual Studio Code.app/Contents
β’ Flutter extension version 2.19.0
[β] Connected device (1 available)
β’ iPhone 8 Plus β’ 071BB9E4-2116-4867-B1FF-7BE5B6363459 β’ ios β’ iOS 12.1 (simulator)
! Doctor found issues in 1 category.
```
| framework,f: scrolling,f: gestures,has reproducible steps,P3,team-framework,triaged-framework,found in release: 3.19,found in release: 3.20 | low | Major |
378,219,833 | opencv | cvtColor for BGR to YUV 4:2:0 returns darker image | <!--
If you have a question rather than reporting a bug please go to http://answers.opencv.org where you get much faster responses.
If you need further assistance please read [How To Contribute](https://github.com/opencv/opencv/wiki/How_to_contribute).
Please:
* Read the documentation to test with the latest developer build.
* Check if other person has already created the same issue to avoid duplicates. You can comment on it if there already is an issue.
* Try to be as detailed as possible in your report.
* Report only one problem per created issue.
This is a template helping you to create an issue which can be processed as quickly as possible. This is the bug reporting section for the OpenCV library.
-->
##### System information (version)
- OpenCV => 3.1
- Operating System / Platform => Ubuntu 16.04 64 bit
- Compiler => gcc version 5.4.0
##### Detailed description
The grayscale image that comes from cvtColor function with COLOR_BGR2YUV_I420 (or COLOR_BGR2YUV_IYUV), which converts RGB to YUV 4:2:0 family, is darker than the grayscale image that comes from COLOR_BGR2GRAY. I think in theory they should be the same because the Y channel is actually the grayscale image. Here is a simple code that I used for testing:
##### Steps to reproduce
```
cv::Mat inputImage=cv::imread("Lenna.png");
cv::Mat gray_BGR2GRAY;
cv::cvtColor(inputImage, gray_BGR2GRAY, cv::COLOR_BGR2GRAY);
imshow("gray_BGR2GRAY", gray_BGR2GRAY);
cv::Mat mat_BGR2YUV_I420;
cv::cvtColor(inputImage, mat_BGR2YUV_I420, cv::COLOR_BGR2YUV_I420);
cv::Mat grayPart_BGR2YUV_I420(mat_BGR2YUV_I420, cv::Rect(0, 0, inputImage.cols, inputImage.rows));
imshow("grayPart_BGR2YUV_I420", grayPart_BGR2YUV_I420);
cv::waitKey(0);
```
for reading the grayscale part I used the answer to the following question:
http://answers.opencv.org/question/100149/how-to-get-y-u-v-from-image/ | category: imgproc,RFC | low | Critical |
378,226,535 | pytorch | Caffe2 install | i'm trying to install Caffes2 by the following commands:
##########
brew install \
automake \
cmake \
git \
gflags \
glog \
python
pip install --user \
future \
numpy \
protobuf \
pyyaml \
six
git clone https://github.com/pytorch/pytorch.git && cd pytorch
git submodule update --init --recursive
python setup.py install
########
but I get an error when running"python setup.py install":
########
Building wheel torch-1.0.0a0+a132a7d
running install
setup.py::run()
running build_deps
setup.py::build_deps::run()
Traceback (most recent call last):
File "setup.py", line 1244, in <module>
rel_site_packages + '/caffe2/**/*.py'
File "/System/Library/Frameworks/Python.framework/Versions/2.7/lib/python2.7/distutils/core.py", line 151, in setup
dist.run_commands()
File "/System/Library/Frameworks/Python.framework/Versions/2.7/lib/python2.7/distutils/dist.py", line 953, in run_commands
self.run_command(cmd)
File "/System/Library/Frameworks/Python.framework/Versions/2.7/lib/python2.7/distutils/dist.py", line 972, in run_command
cmd_obj.run()
File "setup.py", line 724, in run
self.run_command('build_deps')
File "/System/Library/Frameworks/Python.framework/Versions/2.7/lib/python2.7/distutils/cmd.py", line 326, in run_command
self.distribution.run_command(command)
File "/System/Library/Frameworks/Python.framework/Versions/2.7/lib/python2.7/distutils/dist.py", line 972, in run_command
cmd_obj.run()
File "setup.py", line 465, in run
check_pydep('yaml', 'pyyaml')
File "setup.py", line 346, in check_pydep
raise RuntimeError(missing_pydep.format(importname=importname, module=module))
RuntimeError: Missing build dependency: Unable to `import yaml`.
Please install it via `conda install pyyaml` or `pip install pyyaml`
########
then, I tried "pip install pyyaml":
Requirement already satisfied: pyyaml in /Users/Winnie/Library/Python/3.7/lib/python/site-packages (3.13)
########
can any one tell me what happened?
OS X El Capitan
python 2.7.10 | caffe2 | low | Critical |
378,235,731 | vue | Throw error when using interpolation instead of JS expression for slot names | ### What problem does this feature solve?
For this demo:
[https://jsfiddle.net/2os9jken/](https://jsfiddle.net/2os9jken/)
opening the console and running it throws the `Interpolation inside attributes has been removed. Use v-bind or the colon shorthand instead.` error for the `span`, but it should also throw it for the `slot`, as it has the exact same issue for its `name` attribute.
### What does the proposed API look like?
N/A
<!-- generated by vue-issues. DO NOT REMOVE --> | feature request,good first issue,has PR | low | Critical |
378,240,854 | TypeScript | parameter property's modifier may not be followed by newline |
<!-- Please try to reproduce the issue with `typescript@next`. It may have already been fixed. -->
**TypeScript Version:** has been that way since at least 2.4
<!-- Search terms you tried before logging this (so others can find this issue more easily) -->
**Search Terms:**
**Code**
```ts
class Foo {
constructor(public
foo: string) {}
}
```
**Expected behavior:**
Treat is as a parameter property. Or at least document this in the spec (related #28395)
**Actual behavior:**
Treats it as two parameters with a syntax error because of a missing comma.
**Playground Link:** https://agentcooper.github.io/typescript-play/#code/MYGwhgzhAEBiD29oG8BQ1rHgOwgFwCcBXYPeAgCgAciAjEAS2HQ2gDNEAuafAh7AOYBKFAF9UooA
**Related Issues:** <!-- Did you find other bugs that looked similar? -->
| Bug | low | Critical |
378,274,565 | godot | Add "hover" and "pressed" styles to Slider theme options | The HSlider theme class currently offers the following options:
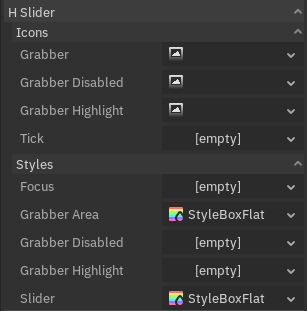
From what I've seen, there is no way to make the Slider StyleBox look different when hovered or pressed, which limits customization possibilities. For those wondering, it's used to represent the "filled" area of the slider (shown in orange below):
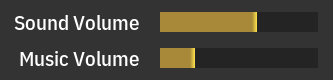
| enhancement,topic:gui | low | Minor |
378,292,428 | pytorch | [caffe2] Modify models in model zoo | I'd like to load the imagenet resnet50 in model zoo and make a change on it. But I can not find any documents or examples of how to do that. A related issues is [https://github.com/pytorch/pytorch/issues/7614](url), but no answers for that yet.
To be specific, I'd like to skip the final fc operator in the original resnet. I can think of two possible ways:
1) Change the pbtxt model file and load the modified model.
As the pbtxt model file is a text file, I can edit it directly. But I can not find how to convert the modified pbtxt file to a pb model file. Another possible way is to load model from the pbtxt file directly, but again I don't find document about that.
2) Load the original model and edit the mode in memory.
This may require APIs to change the model structure of a loaded model.
Does anyone know how to do that?
| caffe2 | low | Minor |
378,297,291 | vscode | Exclude gitignored files from file watcher | I would like an option to disable watching of gitgnored files (i.e. add them to the `files.watcherExclude` option automatically).
I think this makes sense, at least I couldn't think of a use case for watching gitignored files. It might also help with [autorefresh related issues](https://github.com/Microsoft/vscode/issues/42821#issuecomment-436627837) and could make the `files.watcherExclude` option thinner. | feature-request,file-watcher | high | Critical |
378,333,725 | pytorch | Caffe2 Build Static | Is it possible to build caffe2 as static library?
I was trying to add flag STATIC, but it seems a lot of problems with install | caffe2 | low | Minor |
378,351,564 | rust | 2018 idioms: incorrect suggestion for root module and crate of same name | First reported at https://github.com/rust-lang/cargo/issues/6273
this code:
```rust
#![warn(rust_2018_idioms)]
extern crate time as std_time;
pub mod time {
pub fn f() {
println!("{:?}", crate::std_time::now());
}
}
fn main() {}
```
[when compiled](https://play.rust-lang.org/?version=beta&mode=debug&edition=2018&gist=b3d0b4244408b208f6dc9ad9b60f7fa5) gives a warning that, when applied, produces code that doesn't compile | A-lints,E-needs-test,A-suggestion-diagnostics,A-edition-2018 | low | Critical |
378,359,384 | vue | Conditional template not rendering when it has the same slot name | ### Version
2.5.17
### Reproduction link
[https://jsfiddle.net/ad17bgtq](https://jsfiddle.net/ad17bgtq)
### Steps to reproduce
1. Create a component that has a named slot exposing a boolean flag in its scope
2. Consume the component with two templates inside it, each with a v-if using the exposed boolean flag
3. Only the last template written in the order of code is rendered
### What is expected?
All templates using the same slot name should render when their v-if evaluates true.
### What is actually happening?
Only the last template written in the code order is rendered. Any other template with the same slot name will not render even if its v-if permits it.
<!-- generated by vue-issues. DO NOT REMOVE --> | has workaround | medium | Minor |
378,418,790 | go | cmd/compile: reduce generated algs for compiler created array types | There are alot of types algs that seem to be generated due to slicelit initializations:
example from go binary:
```
TEXT type..hash.[11]debug/elf.intName(SB) <autogenerated>
TEXT type..hash.[12]debug/elf.intName(SB) <autogenerated>
TEXT type..hash.[186]debug/elf.intName(SB) <autogenerated>
TEXT type..hash.[20]debug/elf.intName(SB) <autogenerated>
TEXT type..hash.[28]debug/elf.intName(SB) <autogenerated>
TEXT type..hash.[2]debug/elf.intName(SB) <autogenerated>
TEXT type..hash.[3]debug/elf.intName(SB) <autogenerated>
TEXT type..hash.[41]debug/elf.intName(SB) <autogenerated>
TEXT type..hash.[5]debug/elf.intName(SB) <autogenerated>
TEXT type..hash.[6]debug/elf.intName(SB) <autogenerated>
TEXT type..hash.[9]debug/elf.intName(SB) <autogenerated>
```
There are a few other places were the compiler constructs an array type (e.g. non escaping small slice) where algs are likely not needed.
We should vet the compiler for compiler created types (sinit.go, walk.go) that dont need algs and do a SetNoalg where algs are not needed.
Some overzealous application of SetNoalg on new array types in gc seems to remove the above examples and saves ~45kbyte from the go binary. This might be an upper bound because maybe for some cases we will need them.
I have some other ideas/cls to improve algs (or remove them) for go1.13 so happy to work on this.
@josharian @randall77 | Performance,NeedsInvestigation,compiler/runtime | low | Critical |
378,420,123 | TypeScript | Suggestion and codefix to add missing cases to switch | **TypeScript Version:** 3.2.0-dev.20181106
**Code**
```ts
enum E { A, B, C }
function f(x: E) {
switch (x) {
case E.A: return "a";
case E.C: return "c";
}
}
```
**Expected behavior:**
Suggestion on `x` recommending to add a case `case E.B:`, and a codefix which does that (perhaps by adding `case E.B: throw new Error("todo");`).
**Actual behavior:**
No suggestion. | Suggestion,In Discussion,Domain: Quick Fixes | low | Critical |
378,455,675 | rust | Investigate & fix compiler options that print to stdout | Compiler options that print to stdout instead of stderr will break Rustbuild if they are enabled during a bootstrap (see #43855).
We should audit `rustc` for options which print to stdout and change them to print to stderr instead.
As @eddyb [points out](https://github.com/rust-lang/rust/pull/55495#issuecomment-436634290) we should also investigate any `*.stdout` files in the `src/test` directory. | C-enhancement,T-compiler | low | Minor |
378,465,934 | vscode | Add a command to put OS / VS Code info into clipboard | A lot of times I want to open issues directly on GitHub. Now if I want to provide VS Code's info, I need to launch the issue helper, fill in title / body, open GitHub link, copy the info from there.
I would want a command to directly get those informations into the clipboard. | feature-request,workbench-diagnostics,issue-reporter | low | Major |
378,490,244 | pytorch | [caffe2] Adding CUDA operators for generate proposals and NMS layers | ## π Feature
Adding CUDA version for the operator generate proposals + CUDA version of the NMS utils.
## Motivation
Both CUDA layers have been implemented and show significant speed up against their CPU version.
## Pitch
For a Mask-R-CNN network, we see a ~25% end-to-end speed up on training against the same network using the CPU version of those layers. We see an inference speed up of more than 5x.
## Additional context
We've been working with FB engineers on this - The choice to work on those specifics layers + the benchmarking results are part of this work
| caffe2 | low | Minor |
378,532,391 | vscode | Git - Provide task progress | **Request**
It could be a nice feature to show the git progress details in the GIT output console or since Visual Studio even though is 'Code' has the characteristic to being 'Visual', polish the user interface with some indicator.
In the following images there are some suggestions:
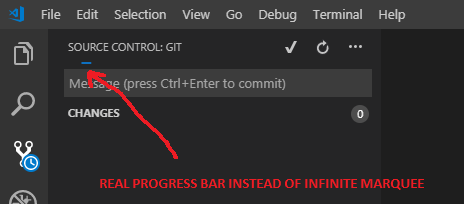
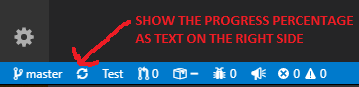
**Use case**
It happen sometime that users have in their own repository some big amount files or maybe the connection slow down during the 'git push', users don't have any idea what is happening or what is the current operation state (it could take even plenty of minutes).
In the example I'have done, this is what is showed actually in VS Code:
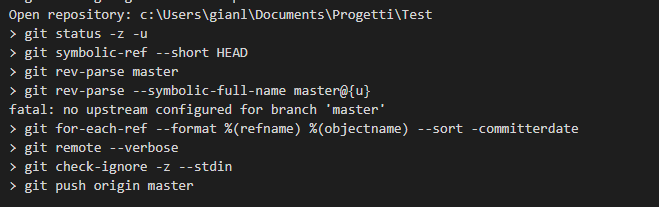
And this is what it can be read from the git executable output:

**Advantages**
Users that have this kind of experience can think that the git integration (that is already amazing in VS Code) is not working very good because the operations appears 'blocked'. So they give up and jump on the CLI for the daily usage of git. With some nice improvements like I showed they wouldn't do it.
**Technical inspection**
I inspected the main source code file about the default GIT extension:
[https://github.com/Microsoft/vscode/blob/master/extensions/git/src/git.ts](https://github.com/Microsoft/vscode/blob/master/extensions/git/src/git.ts)
A nice implementation could be extract more information on the 'git commands output' parsing, ex. Operation 'N' of 'M' and 'Percentage', expose it and use on higher layer of Visual Studio Code.
Waiting for a your feedback.
Thank you.
| feature-request,git | medium | Major |
378,536,976 | go | net: only js/wasm returns an error on writes to closed connections | If a localhost connection is closed by the peer, all other GOOS seem to allow writes to happen without error, while js/wasm returns `write tcp 127.0.0.1:XXX->127.0.0.1:1: Socket is not connected` with XXX changing.
I admit it does feel like something that should return an error, and I don't know if there is a precise requirement to do otherwise, but js being the only GOOS to do it is very inconsistent, and not even NaCl on the playground with its fictitious network returns an error.
This came up because js/wasm were the only broken TryBots in some crypto/tls CL: https://storage.googleapis.com/go-build-log/ca02321c/js-wasm_d63aa3d8.log
https://play.golang.org/p/lRhq44NJXTr (this specific program will actually panic on js/wasm due to #28649, but it proves the `log.Print` is being reached)
/cc @neelance | NeedsInvestigation,arch-wasm,OS-JS | low | Critical |
378,580,517 | scrcpy | Media Control Shortcuts | I think a useful addition would be to support Play/Pause, Next and Previous key support.
When connecting a USB or Bluetooth keyboard with media keys to a phone the buttons work as expected, but they don't seem to be passed through to the device when using scrcpy.
I'm not sure if it makes more sense to have a separate shortcut or to actually listen to the media keys themselves. | feature request | low | Minor |
378,581,403 | go | cmd/go: go test -cover doesn't collect the coverage of a second call to m.Run | <!-- Please answer these questions before submitting your issue. Thanks! -->
### What version of Go are you using (`go version`)?
<pre>
$ go version
go1.10.3 darwin/amd64
</pre>
### Does this issue reproduce with the latest release?
### What operating system and processor architecture are you using (`go env`)?
<details><summary><code>go env</code> Output</summary><br><pre>
$ go env
GOARCH="amd64"
GOBIN=""
GOCACHE="/Users/wangheng/Library/Caches/go-build"
GOEXE=""
GOHOSTARCH="amd64"
GOHOSTOS="darwin"
GOOS="darwin"
GOPATH="/Users/wangheng/go"
GORACE=""
GOROOT="/usr/local/Cellar/go/1.10.3/libexec"
GOTMPDIR=""
GOTOOLDIR="/usr/local/Cellar/go/1.10.3/libexec/pkg/tool/darwin_amd64"
GCCGO="gccgo"
CC="clang"
CXX="clang++"
CGO_ENABLED="1"
CGO_CFLAGS="-g -O2"
CGO_CPPFLAGS=""
CGO_CXXFLAGS="-g -O2"
CGO_FFLAGS="-g -O2"
CGO_LDFLAGS="-g -O2"
PKG_CONFIG="pkg-config"
GOGCCFLAGS="-fPIC -m64 -pthread -fno-caret-diagnostics -Qunused-arguments -fmessage-length=0 -fdebug-prefix-map=/var/folders/qf/_x6np3lx7qb9sn_0ymk53fqr0000gn/T/go-build681943823=/tmp/go-build -gno-record-gcc-switches -fno-common"
</pre></details>
### What did you do?
I wrote a very simple code to describe the issue. Please see the go files I attached. It seems 'golang cover' only counted the first main thread coverage and cast the coverage of second m.Run() away.
β first go test -cover
PASS
coverage: 0.0% of statements
PASS
ok go/test/first 0.013s
<!--
If possible, provide a recipe for reproducing the error.
A complete runnable program is good.
A link on play.golang.org is best.
-->
### What did you expect to see?
### What did you see instead?
| help wanted,NeedsInvestigation | low | Critical |
378,612,009 | flutter | Allow SliverAppBar to have other slivers laid out below it | AppBar has a `floating` property and `pinned` property. Currently when both of these are set to true, and the AppBar is made transparent, the AppBar still pushes content below it (in the y-axis), leaving an empty box beneath the AppBar (in the z-axis).
Either, the behavior of setting `floating` and `pinned` to `true`, when combined with transparency, should cause content to expand up and beneath the AppBar, or we need to implement this behavior with a flag because developers need it. | c: new feature,framework,f: material design,f: scrolling,P3,team-design,triaged-design | low | Major |
378,636,100 | opencv | cvTriangulatePoints() supports only two-view triangulation | **Feature request** to add multi-view triangulation (more than 2 views) to `cvTriangulatePoints()`. | priority: low | low | Minor |
378,647,028 | pytorch | caffe2 c++ load pb model fail | Hi everyone, i convert shufflenet v2 to caffe2 pb model, which correct run in python caffe2, but when i load pb model in caffe2 c++, it can't generate predictor, my code is as follows:
`namespace caffe2 {
void run() {
std::cout << std::endl;
std::cout << "## Caffe2 Loading Pre-Trained Models Tutorial ##" << std::endl;
std::cout << "https://caffe2.ai/docs/zoo.html" << std::endl;
std::cout << "https://caffe2.ai/docs/tutorial-loading-pre-trained-models.html"
<< std::endl;
std::cout << "https://caffe2.ai/docs/tutorial-image-pre-processing.html"
<< std::endl;
std::cout << std::endl;
if (!std::ifstream(FLAGS_init_net).good() ||
!std::ifstream(FLAGS_predict_net).good()) {
std::cerr << "error: Squeezenet model file missing: "
<< (std::ifstream(FLAGS_init_net).good() ? FLAGS_predict_net
: FLAGS_init_net)
<< std::endl;
std::cerr << "Make sure to first run ./script/download_resource.sh"
<< std::endl;
return;
}
if (!std::ifstream(FLAGS_file).good()) {
std::cerr << "error: Image file missing: " << FLAGS_file << std::endl;
return;
}
if (!std::ifstream(FLAGS_classes).good()) {
std::cerr << "error: Classes file invalid: " << FLAGS_classes << std::endl;
return;
}
std::cout << "init-net: " << FLAGS_init_net << std::endl;
std::cout << "predict-net: " << FLAGS_predict_net << std::endl;
std::cout << "file: " << FLAGS_file << std::endl;
std::cout << "size: " << FLAGS_size << std::endl;
std::cout << std::endl;
// >>> img =
// skimage.img_as_float(skimage.io.imread(IMAGE_LOCATION)).astype(np.float32)
auto image = cv::imread(FLAGS_file); // CV_8UC3
std::cout << "image size: " << image.size() << std::endl;
// scale image to fit
cv::Size scale(std::max(FLAGS_size * image.cols / image.rows, FLAGS_size),
std::max(FLAGS_size, FLAGS_size * image.rows / image.cols));
cv::resize(image, image, scale);
std::cout << "scaled size: " << image.size() << std::endl;
// crop image to fit
cv::Rect crop((image.cols - FLAGS_size) / 2, (image.rows - FLAGS_size) / 2,
FLAGS_size, FLAGS_size);
image = image(crop);
std::cout << "cropped size: " << image.size() << std::endl;
// convert to float, normalize to mean 128
image.convertTo(image, CV_32FC3, 1.0, -128);
std::cout << "value range: ("
<< *std::min_element((float *)image.datastart,
(float *)image.dataend)
<< ", "
<< *std::max_element((float *)image.datastart,
(float *)image.dataend)
<< ")" << std::endl;
// convert NHWC to NCHW
vector<cv::Mat> channels(3);
cv::split(image, channels);
std::vector<float> data;
for (auto &c : channels) {
data.insert(data.end(), (float *)c.datastart, (float *)c.dataend);
}
std::vector<TIndex> dims({1, image.channels(), image.rows, image.cols});
TensorCPU tensor(dims, data, NULL);
// Load Squeezenet model
NetDef init_net, predict_net;
// >>> with open(path_to_INIT_NET) as f:
CAFFE_ENFORCE(ReadProtoFromFile(FLAGS_init_net, &init_net));
std::cout <<">>>>>>>>>>>>>>>"<< std::endl;
// >>> with open(path_to_PREDICT_NET) as f:
CAFFE_ENFORCE(ReadProtoFromFile(FLAGS_predict_net, &predict_net));
std::cout <<">>>>>>>>>>>>>>>"<< std::endl;
// >>> p = workspace.Predictor(init_net, predict_net)
Workspace workspace("tmp");
CAFFE_ENFORCE(workspace.RunNetOnce(init_net));
auto input = workspace.CreateBlob("data")->GetMutable<TensorCPU>();
input->ResizeLike(tensor);
input->ShareData(tensor);
CAFFE_ENFORCE(workspace.RunNetOnce(predict_net));
std::cout <<">>>>>>>>>>>>>>>"<< std::endl;
// >>> results = p.run([img])
auto &output_name = predict_net.external_output(0);
auto output = workspace.GetBlob(output_name)->Get<TensorCPU>();
// sort top results
const auto &probs = output.data<float>();
std::vector<std::pair<int, int>> pairs;
for (auto i = 0; i < output.size(); i++) {
if (probs[i] > 0.01) {
pairs.push_back(std::make_pair(probs[i] * 100, i));
}
}
std::sort(pairs.begin(), pairs.end());
std::cout << std::endl;
// read classes
std::ifstream file(FLAGS_classes);
std::string temp;
std::vector<std::string> classes;
while (std::getline(file, temp)) {
classes.push_back(temp);
}
// show results
std::cout << "output: " << std::endl;
for (auto pair : pairs) {
std::cout << " " << pair.first << "% '" << classes[pair.second] << "' ("
<< pair.second << ")" << std::endl;
}
}
} // namespace caffe2
`
the error is
terminate called after throwing an instance of 'caffe2::EnforceNotMet'
what(): [enforce fail at operator.cc:42] blob != nullptr. op Conv: Encountered a non-existing input blob: 0
anyone help me ? | caffe2 | low | Critical |
378,653,656 | pytorch | depthwise convolution are slow on cpu | I try to use depthwise convolution to reduce parameters of my model. However I found depthwise convolutions are slow on cpu, just 4x~5x than normal 3x3 convolution, while input_channel and output channel are 256. Is there any way to speed up the process? My version is pytorch 0.4.1.
cc @VitalyFedyunin @ngimel | module: performance,module: cpu,module: convolution,triaged | low | Major |
378,656,921 | go | x/build/cmd/gopherbot: add command to ping owners of the package | It would be good to ping owners of the package in the ticket without remembering who is the owner of it. What if one could say:
```
@gopherbot please ping owners
@gopherbot please ping owners to critique the solution
```
And then gopher would produce something like:
```
@name has asked @firstowner @secondowner to review this issue
@name has asked @firstowner @secondowner to critique the solution
``` | Builders,FeatureRequest | low | Minor |
378,687,621 | react-native | SafeAreaView doesn't respect `padding` property in style | ## Environment
```
React Native Environment Info:
System:
OS: macOS 10.14
CPU: x64 Intel(R) Core(TM) i5-4258U CPU @ 2.40GHz
Memory: 34.00 MB / 8.00 GB
Shell: 3.2.57 - /bin/bash
Binaries:
Node: 9.7.1 - ~/.nvm/versions/node/v9.7.1/bin/node
Yarn: 1.2.1 - /usr/local/bin/yarn
npm: 6.4.1 - ~/.nvm/versions/node/v9.7.1/bin/npm
Watchman: 4.7.0 - /usr/local/bin/watchman
SDKs:
iOS SDK:
Platforms: iOS 12.0, macOS 10.14, tvOS 12.0, watchOS 5.0
Android SDK:
Build Tools: 21.1.2, 22.0.1, 23.0.1, 23.0.3, 25.0.0, 25.0.2, 26.0.1, 26.0.2, 27.0.1, 27.0.3, 28.0.2, 28.0.3
API Levels: 17, 18, 19, 22, 23, 25, 26, 27, 28
IDEs:
Android Studio: 3.2 AI-181.5540.7.32.5056338
Xcode: 10.0/10A254a - /usr/bin/xcodebuild
npmPackages:
react: 16.6.0 => 16.6.0
react-native: ^0.57.3 => 0.57.3
npmGlobalPackages:
react-native-cli: 2.0.1
```
## Description
Applying `padding` to SafeAreaView's style doesn't work.
## Reproducible Demo
https://snack.expo.io/@danielmartin/c2FmZW
| Component: SafeAreaView,Component: View,Bug,Needs: Repro | high | Critical |
378,710,070 | godot | Poor Light2D performance on Radeon GPU | <!-- Please search existing issues for potential duplicates before filing yours:
https://github.com/godotengine/godot/issues?q=is%3Aissue
-->
**Godot version:**
3.1 c025f526c
**OS/device including version:**
Windows 10: AMD Radeon RX Vega 64


Windows 7: AMD Radeon HD 6870


MacOSX: Radeon Pro 450 2G
**Issue description:**
I got multiple reports from players claiming poor performance. A common factor seems to be use of Radeon cards. According to spec, these cards should be able to run my game >60FPS. Actual real life performance:
AMD Radeon RX Vega 64: ~10FPS
AMD Radeon HD 6870: ~1FPS
AMD Radeon Pro 450 2G: ~2FPS
**Steps to reproduce:**
Grab a [demo from steam](https://store.steampowered.com/app/846030/V_Rings_of_Saturn/), start game, fly to the left to trigger end-demo sequence.
Might be related to #4151 - i do use lot of Light2D in that scene.
| platform:windows,topic:rendering,confirmed,topic:thirdparty,topic:2d,performance | medium | Major |
378,710,437 | TypeScript | Extend tsserver interface to allow refactoring failure reporting | <!-- π¨ STOP π¨ π¦π§π’π£ π¨ πΊπ»πΆπ· π¨
Half of all issues filed here are duplicates, answered in the FAQ, or not appropriate for the bug tracker. Please read the FAQ first, especially the "Common Feature Requests" section.
-->
## Search Terms
refactoring report failure fail
<!-- List of keywords you searched for before creating this issue. Write them down here so that others can find this suggestion more easily -->
## Suggestion
I would like a way to report refactoring failures similar to rename fail report ("You cannot rename this element").
This change improves transparency.
Edit: This might imply changes in VS Code language server extension as well. I'm not familiar with it though.
<!-- A summary of what you'd like to see added or changed -->
## Use Cases
This would be useful to allow refactorings to initially show the refactoring, but when invoking it, an error message should be shown, instead of just not showing the refactoring. This would be confusing to the user.
<!--
What do you want to use this for?
What shortcomings exist with current approaches?
-->
## Examples
Let's say we want to inline a variable, that is not initialized. This should not be possible. But it is still useful to show the refactoring initially, since the user may be confused why the option doesn't show up.
Then, if the user wants to invoke the refactoring, they get the error message "Cannot inline uninitialized variable."
```
let notInitialized; // should not allow inlining, but tell user why
foo(notInitialized);
```
<!-- Show how this would be used and what the behavior would be -->
## Checklist
My suggestion meets these guidelines:
* [*] This wouldn't be a breaking change in existing TypeScript / JavaScript code
* [*] This wouldn't change the runtime behavior of existing JavaScript code
* [*] This could be implemented without emitting different JS based on the types of the expressions
* [*] This isn't a runtime feature (e.g. new expression-level syntax)
| Suggestion,In Discussion,API,Domain: TSServer | low | Critical |
378,714,389 | tensorflow | Move autograph into a separate package and into a separate repo | ### System information
- **Have I written custom code (as opposed to using a stock example script provided in TensorFlow)**: no
- **OS Platform and Distribution (e.g., Linux Ubuntu 16.04)**: any
- **Mobile device (e.g. iPhone 8, Pixel 2, Samsung Galaxy) if the issue happens on mobile device**: n/a
- **TensorFlow installed from (source or binary)**: any
- **TensorFlow version (use command below)**: any
- **Python version**: any
- **Bazel version (if compiling from source)**: any
- **GCC/Compiler version (if compiling from source)**: any
- **CUDA/cuDNN version**: any
- **GPU model and memory**: any
- **Exact command to reproduce**: n/a
### Describe the problem
I guess autograph should be moved into a separate package and repo. Because I guess it may be possible to add support of other computation graph frameworks there.
| stat:awaiting tensorflower,type:feature,WIP,comp:autograph | low | Major |
378,718,665 | TypeScript | Suggestion: compiler option to validate "empty imports" | <!-- π¨ STOP π¨ π¦π§π’π£ π¨ πΊπ»πΆπ· π¨
Half of all issues filed here are duplicates, answered in the FAQ, or not appropriate for the bug tracker. Please read the FAQ first, especially the "Common Feature Requests" section.
-->
## Search Terms
<!-- List of keywords you searched for before creating this issue. Write them down here so that others can find this suggestion more easily -->
empy import cannot find module symbols side deffect
## Suggestion
Current behaviour: given an [empty import](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/import#Import_a_module_for_its_side_effects_only) (an import that has side effects and/or does not import any symbols):
``` ts
import './abc';
```
If the module does not exist, there will be no error.
This is unlike other (non-empty) imports, which will error for non-existent modules:
``` ts
import 'foo' from ./abc';
```
I understand this is intended behaviour as per https://github.com/Microsoft/TypeScript/issues/20534#issuecomment-350846017.
I would like to suggest a compiler option for people to opt-in to the stricter behaviour. This could also be added to the `strict` compiler option.
## Checklist
My suggestion meets these guidelines:
* [x] This wouldn't be a breaking change in existing TypeScript / JavaScript code
* [x] This wouldn't change the runtime behavior of existing JavaScript code
* [x] This could be implemented without emitting different JS based on the types of the expressions
* [x] This isn't a runtime feature (e.g. new expression-level syntax)
| Suggestion,In Discussion | low | Critical |
378,769,306 | TypeScript | Better handling for manually created source files and compiler APIs | ## Search Terms
type checker source file cannot ready property 'members' of undefined
## Suggestion
When a user of the compiler API manually creates a source file with `ts.createSourceFile` instead of retrieving it from their `program`, and then asks the `program` for type inference on a contained node, this can crash. See https://github.com/Microsoft/TypeScript/issues/8136 / https://github.com/vuejs/vue-cli/issues/2712 / https://github.com/angular/tsickle/issues/151 / https://github.com/general-language-syntax/TS-GLS/issues/39.
It seems like one of three interpretations might be best:
* This is explicitly unsupported behavior, but for the sake of performance & simplicity, no checks should happen
* This is explicitly unsupported behavior, and a more explicit error should be thrown
* This should become supported behavior, and the program should dynamically create source files as requested
## Use Cases
Auto-generated TypeScript files, such as `.vue` snippets, still want access to a type checker, such as for TSLint rules.
## Examples
https://github.com/palantir/tslint/issues/4273
## Checklist
My suggestion meets these guidelines:
* [x] This wouldn't be a breaking change in existing TypeScript / JavaScript code
* [x] This wouldn't change the runtime behavior of existing JavaScript code
* [x] This could be implemented without emitting different JS based on the types of the expressions
* [x] This isn't a runtime feature (e.g. new expression-level syntax)
| Suggestion,Needs Proposal,API | low | Critical |
378,804,381 | go | net: Resolve{TCP,UDP,IP}Addr returns IPv4 address on host without IPv4 connectivity | ### What version of Go are you using (`go version`)?
<pre>
$ go version
go version go1.11rc2 linux/amd64
</pre>
### Does this issue reproduce with the latest release?
Yes
### What operating system and processor architecture are you using (`go env`)?
Linux. The issue doesn't depend on CPU arch and has been reproduced on
ARMv5 and x86-64.
### What did you do?
A minimal repro is this program:
```go
package main
import (
"log"
"net"
)
func main() {
r, _ := net.ResolveUDPAddr("udp", "ping.sunet.se:0") // IPv4 and IPv6 records
log.Printf("Result of ResolveUDPAddr: %v", r)
r2, _ := net.ResolveIPAddr("ip", "ping.sunet.se") // IPv4 and IPv6 records
log.Printf("Result of ResolveIPAddr: %v", r2)
r3, _ := net.ResolveIPAddr("ip", "ipv6.sunet.se") // Only IPv6 record
log.Printf("Result of ResolveIPAddr: %v", r3)
}
```
This shows the behavior of ResolveUDPAddr and ResolveIPAddr with dual-stacked
hosts and a IPv6 only host as the last entry. This also applies to ResolveTCPAddr.
### What did you expect to see?
On hosts that have an IPv6 and an IPv4 address, the IPv6 address is preferred
on hosts that has IPv6 only connectivity.
In the program below, the output **should** be something like this on an IPv6-only system:
```
[bluecmd]$ ./ipv6_dial
2018/11/08 16:22:56 Result of ResolveUDPAddr: 2001:6b0:7::14
2018/11/08 16:22:56 Result of ResolveIPAddr: 2001:6b0:7::14
2018/11/08 16:22:56 Result of ResolveIPAddr: 2001:6b0:7::14
```
### What did you see instead?
This is the outcome on an IPv6 only system:
```
[bluecmd]$ sudo ip ro
[bluecmd]$ sudo ip -6 ro
[..]
default via fe80::200:5eff:fe00:265 dev eth0 proto ra metric 100 pref medium
```
Yet ResolveIPAddr prefers IPv4:
```
[bluecmd]$ ./ipv6_dial
2018/11/08 16:22:56 Result of ResolveUDPAddr: 192.36.125.18:0
2018/11/08 16:22:56 Result of ResolveIPAddr: 192.36.125.18
2018/11/08 16:22:56 Result of ResolveIPAddr: 2001:6b0:7::14
```
This is also not dependent on CGO.
For more logs, see [here](https://gist.github.com/bluecmd/7c847fcd22d50bc8ed6f078136c4a6c8).
### Extra information
Problematic code is most likely this part of ipsock.go:
https://github.com/golang/go/blob/7da1f7addf54e55c7b40c1a7bffe5a64989154d8/src/net/ipsock.go#L73-L98
My reading is that the code returns IPv4 unless IPv6 was explicitly asked for.
If the host is IPv6 only, then that host is picked on the fallback return in first().
I would suggest changing the code to not prefer IPv4 addresses if there is no IPv4 connectivity. | NeedsInvestigation | medium | Major |
378,834,662 | go | cmd/compile: various low level x86 instruction generation improvements | While reading (to much) go generated assembly code I picked up a few x86 code sequences that seemed sub optimal. I do not remember where I had spotted each of them and some might just come from my imagination, compiler optimization guides or from outside the std library.
Instead of creating an issue per possibility here is a list of some possible low level performance improvements. Note that this does not mean they are common and therefore worth introducing. That can be evaluated. However these can serve to spark ideas for other improvements and for new compiler contributors to try out adding ssa optimization rules or codegen improvements and benchmarking their effects and frequency. UPDATE: CLs should make sure to include statistics/examples of use in std lib and/or generally when introducing optimizations.
Current assembly gc and gccgo create can be quickly checked with https://godbolt.org/.
Given the low level nature there might be oversights in what is possible and whether they are size or performance improvements. As always needs benchmarks and tests.
Many of them should be considered as examples for more general optimizations.
The list:
1. no baseless lea:
There is no lea without a base and only ```index*operandsize``` resulting in ```x+x+x+x``` being compiled to ```leaq+addq``` instead of a single ```leaq[0+x*4]``` with some more combination rules.
2. to many imul
```x*x*x*x``` is compiled as 3 ```imuls``` when 2 are sufficient.
3. set all bits in a register (UPDATE: not generally worth it due to false dependency)
Instead of ```MOVQ $-0x1, Reg``` use ```ORQ $-0x1, Reg``` which is shorter by ~4 bytes for 64bit ints but may create a false dependency as noted by @randall77.
4. comparing modulo
```x % 2 == 0``` can be ```andl $1, %eax, testq %rax, %rax``` (or ```btl $0, AX``` ...) instead of ```shift+shift+add+shift+shift+cmp```
5. instead of add use subtract for some powers of 2
```sub -128``` is shorter than ```add 128```. (watch out that flags are not used)
6. for some powers of 2 less equal is better than less of a bit more
```x < 128``` encodes to ```CMPQ $0x80, AX; JG``` which is larger than ```CMPQ $0x7f, AX; JGE```. Should work similar for other comparisons encoding of constants.
7. unsigned division with int
If it is known the int divisor is positive instead of ```CQO+IDIV``` a ```XOR+DIV``` could be used.
8. optimize modulo with shifts that produce power of 2
```var x,y uint; x % (1<<y)``` can be replaced with ```x & ((1<<y)-1)```.
@josharian @randall77 @TocarIP @Quasilyte | Suggested,Performance,help wanted,NeedsInvestigation,compiler/runtime | low | Major |
378,848,736 | go | x/build/cmd/pubsubhelper: use cloud pubsub for Gerrit instead of email | When I wrote pubsubhelper, Gerrit didn't support Cloud Pub Sub, so we had to parse emails to get live notifications out of Gerrit.
But @andybons pointed out that now it does, so we should move to it.
Low priority, but would be a nice cleanup.
| Builders | low | Major |
378,899,000 | rust | UdpSocket receive to short buffer behaves differently on Unix and Windows | On Unix receiving to a buffer shorter than the incoming payload silently truncates the read. On Windows the read is completed but an error is returned. Not being that familiar with Windows I found this behaviour surprising.
The following comment in [`sys/windows/net.rs`](https://github.com/rust-lang/rust/blob/1d834550d54e4c5211f03f877c1756425f24be98/src/libstd/sys/windows/net.rs#L204-L205) suggests that making Unix and Windows behave the same is (at times) a goal of the standard library:
```
// On unix when a socket is shut down all further reads return 0, so we
// do the same on windows to map a shut down socket to returning EOF.
```
If it is I'd like to propose that `WSAEMSGSIZE` be absorbed so that both platforms behave the same. | T-libs-api,C-bug,A-io | medium | Critical |
378,907,252 | pytorch | [caffe2] test depthwise3x3_conv_op_test fails to run | ## π Bug
The caffe2 test `depthwise3x3_conv_op_test` fails to run when using caffe2 git master in a cpu-only build (with `USE_CUDA:BOOL='OFF'`):
```
Start 84: depthwise3x3_conv_op_test
84/91 Test #84: depthwise3x3_conv_op_test ...............***Failed 0.55 sec
Start 85: common_subexpression_elimination_test
...
99% tests passed, 1 tests failed out of 91
Total Test time (real) = 249.62 sec
The following tests FAILED:
84 - depthwise3x3_conv_op_test (Failed)
Errors while running CTest
make: *** [Makefile:86: test] Error 8
```
## To Reproduce
Steps to reproduce the behavior:
1. mkdir -p build
1. cd build
1. [cmake options](https://bpaste.net/show/ca5a7eb99368)
1. make
1. make test
<!-- If you have a code sample, error messages, stack traces, please provide it here as well -->
## Expected behavior
The `depthwise3x3_conv_op_test` test should pass.
## Environment
- PyTorch Version (e.g., 1.0): git master
- OS (e.g., Linux): Arch Linux x86_64
- How you installed PyTorch (`conda`, `pip`, source): source
- Build command you used (if compiling from source): already shown above
- Python version: 3.7.1
- CUDA/cuDNN version: disabled in build
- GPU models and configuration: disabled in build
- Any other relevant information: compiler is gcc 8.2.1
## Additional context
- All tests passes when using caffe2 git master with `USE_CUDA:BOOL='ON'`
- All tests passes when using caffe2 1.0rc1 with `USE_CUDA:BOOL='ON'`
- Almost all tests fails when using caffe2 1.0rc1 with `USE_CUDA:BOOL='OFF'`
| caffe2 | low | Critical |
378,915,483 | electron | Add event that can be used to listen for mouse and scroll events on a <webview> | **Is your feature request related to a problem? Please describe.**
As of Electron 3.0.0 beta 6 mouse, keyboard, and scroll events and the like no longer are able to be listened for. #14258 , however as seen in that issue "bpasero" used the "before-input-event" event to create an emulated Keypress. As there already is an input event.
**Describe the solution you'd like**
i'd suggest that there be a "before-mouse-event" and "before-scroll-event" so that there can be consistent solution to fix the regression.
| enhancement :sparkles: | low | Major |
378,919,541 | godot | Window width affects directional shadow resolution (fixed in `master`) | **Godot version:**
Affects both 3.0.6 and Official 3.1 Alpha 2
**OS/device including version:**
Linux Mint 19 - 64bit
Nvidia GeForce GTX 1060 on driver version: 396.54
**Issue description:**
For some reason the directional shadow resolution decreases as the window width increases, and increases as the width decreases.
Happens in the editor window and also in a running game.
[Video](https://github.com/godotengine/godot/files/2563615/what.flv.zip)
I'm assuming this isn't intended behaviour?
OmniLight and SpotLight do not seem affected.
**Steps to reproduce:**
1. Set up a scene with meshes and a Directional Light with shadows enabled.
2. Decrease and increase the width of the 3D scene window and observe shadows. | bug,topic:rendering,confirmed,topic:3d | low | Major |
378,920,700 | bitcoin | Can't configure bitocoind to only send tx via Tor but receive clearnet transactions | <!-- Describe the issue -->
There does not seen to be a way to enable onlynet=onion for sending only and regular clearnet for receiving only.
<!--- What behavior did you expect? -->
I expected that onlynet=onion would mean outbound connections from only onion nodes. This sort of works but I need to manually add nodes and then if a node is not open also to the outside I am in an isolate Bitcoin network. I also do not seem to be getting any inbound connections with onlynet=onion is this because my IP is always different under Tor and I need to post my .onion domain somewhere for people to find?
<!--- What was the actual behavior (provide screenshots if the issue is GUI-related)? -->
<!--- How reliably can you reproduce the issue, what are the steps to do so? -->
<!-- What version of Bitcoin Core are you using, where did you get it (website, self-compiled, etc)? -->
0.17
<!-- What type of machine are you observing the error on (OS/CPU and disk type)? -->
Ubuntu 18.04
<!-- Any extra information that might be useful in the debugging process. -->
<!--- This is normally the contents of a `debug.log` or `config.log` file. Raw text or a link to a pastebin type site are preferred. -->
| Feature,P2P | low | Critical |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.