id
int64 393k
2.82B
| repo
stringclasses 68
values | title
stringlengths 1
936
| body
stringlengths 0
256k
β | labels
stringlengths 2
508
| priority
stringclasses 3
values | severity
stringclasses 3
values |
---|---|---|---|---|---|---|
375,013,234 | pytorch | DataLoader num_workers > 0 causes CPU memory from parent process to be replicated in all worker processes | # Editor note: There is a known workaround further down on this issue, which is to NOT use Python lists, but instead using something else, e.g., torch.tensor directly. See https://github.com/pytorch/pytorch/issues/13246#issuecomment-905703662 . You can use a numpy array, but it only fixes the issue for the `fork` start method. See https://github.com/pytorch/pytorch/issues/13246#issuecomment-1364587359 for more details
## π Bug
CPU memory will leak if the DataLoader `num_workers > 0`.
## To Reproduce
Run the following snippet:
```
from torch.utils.data import Dataset, DataLoader
from PIL import Image
from torchvision import transforms
import os
class DataIter(Dataset):
def __init__(self):
path = "path/to/data"
self.data = []
for cls in os.listdir(path):
for img in os.listdir(os.path.join(path, cls)):
self.data.append(os.path.join(path, cls, img))
def __len__(self):
return len(self.data)
def __getitem__(self, idx):
with Image.open(self.data[idx]) as img:
img = img.convert('RGB')
return transforms.functional.to_tensor(img)
train_data = DataIter()
train_loader = DataLoader(train_data, batch_size=300,
shuffle=True,
drop_last=True,
pin_memory=False,
num_workers=18)
for i, item in enumerate(train_loader):
if i % 200 == 0:
print(i)
```
## Expected behavior
CPU memory will gradually start increasing, eventually filling up the whole RAM. E.g., the process starts with around 15GB and fills up the whole 128GB available on the system.
When the `num_workers=0`, RAM usage is constant.
## Environment
```
PyTorch version: 1.0.0.dev20181028
Is debug build: No
CUDA used to build PyTorch: 9.0.176
OS: Ubuntu 16.04.4 LTS
GCC version: (Ubuntu 5.4.0-6ubuntu1~16.04.10) 5.4.0 20160609
CMake version: version 3.5.1
Python version: 3.5
Is CUDA available: Yes
CUDA runtime version: 9.0.176
GPU models and configuration:
GPU 0: GeForce GTX 1080 Ti
GPU 1: GeForce GTX 1080 Ti
GPU 2: GeForce GTX 1080 Ti
Nvidia driver version: 390.67
cuDNN version: Probably one of the following:
/usr/lib/x86_64-linux-gnu/libcudnn.so.7.1.4
Versions of relevant libraries:
[pip] Could not collect
[conda] Could not collect
PIL.__version__
'5.3.0'
```
## Additional info
There are around 24 million images in the dataset and all image paths are loaded into a single list as presented in the above code snippet.
I have also tried multiple Pytorch (0.4.0 and 0.4.1) versions and the effect is the same.
cc @ezyang @gchanan @zou3519 @bdhirsh @jbschlosser @anjali411 @SsnL @VitalyFedyunin @ejguan | high priority,module: dependency bug,module: multiprocessing,module: dataloader,module: molly-guard,module: memory usage,triaged | high | Critical |
375,013,958 | flutter | [Feature Request][CLI] Include setup for Firebase in Flutter CLI | As developer I want to have the setup for firebase connection automated and baked inside Flutter CLI, something like:
`flutter firebase`
During that, all steps from Firebase Console in "Add Firebase to your Android/iOS app" will be added automatically to the app. | c: new feature,tool,P3,team-tool,triaged-tool | low | Major |
375,034,783 | neovim | cannot delete function if a:000 is referenced externally | Given `t-funcref.vim`:
```vim
let s:q = []
function! F1(...)
call add(s:q, [a:000])
endfunction
" Needs two calls.
call F1({})
call F1({})
" Does not work: Cannot delete function F2: It is being used internally
delfunction F1
q
```
Running `nvim -u t-funcref.vim` results in:
```
Error detected while processing β¦/t-funcref.vim:
line 12:
Cannot delete function F1: It is being used internally
```
This happens via https://github.com/neovim/neovim/blob/cf93b5e9f9eea1b08ca8d7cb124265867b2f3bf9/src/nvim/eval.c#L21114-L21118, which is not used in Vim.
Adding this check to Vim produces the same error there then.
So this is a problem also in Vim, but it does not make the `delfunction` fail.
The check in Neovim was added early on in 4b98ea1e8 already, and later adjusted/fixed in b7dab423e.
NVIM v0.3.2-755-gcf93b5e9f | vimscript | low | Critical |
375,078,545 | puppeteer | Web push notification | How I can subscribe, handle and click on push notification? | feature,chromium,confirmed | medium | Critical |
375,091,182 | rust | rustdoc: blanket impls not showing traits with sufficient indirection? | In the gtk-rs docs, [the page for `Clipboard`](http://gtk-rs.org/docs/gtk/struct.Clipboard.html) doesn't show either the [`ClipboardExt`](http://gtk-rs.org/docs/gtk/trait.ClipboardExt.html) or [`ClipboardExtManual`](http://gtk-rs.org/docs/gtk/prelude/trait.ClipboardExtManual.html) traits, despite both of those traits being available on `Clipboard`.
I *think* this has something to do with the way those traits are implemented. Both extension traits are implemented on `T: IsA<Clipboard>`, where `IsA<T>` is generically implemented for all `<T>`. The `IsA<T>` impl shows up on the `Clipboard` page, but the extension traits do not. Is there a maximum amount of indirection that the trait checker we're using can do? These traits are both usable from regular code.
cc @GuillaumeGomez | T-rustdoc,A-synthetic-impls,E-needs-mcve | low | Minor |
375,094,459 | create-react-app | Errors in IE11 when importing polyfills using es-abstract. | ### Is this a bug report?
Yes
If you import `react-app-polyfill/ie11` before importing any shim/polyfill using `es-abstract` lib, you get an error in IE11 (possibly other browsers as well). Some example polyfills: `object.entries, array-includes, object.values`
Error: `object doesn't support property or method Symbol(Symbol.iterator).9_<some-hash>`
Location: `es-abstract` -> GetIntrinsic.js at line 56.
Workaround: import react-app-polyfill/ie11 after importing all shims/polyfills using `es-abstract`.
### Environment
IE11, presumably anything IE.
### Steps to Reproduce
1. Create new app
2. in index.js, on line 1, `import react-app-polyfill/ie11`
3. in index.js, on line 2, `import objectValues from 'object.values/polyfill'`
4. Open your app in IE11.
### Expected Behavior
App would run.
### Actual Behavior
App crashes. | issue: needs investigation | low | Critical |
375,164,712 | vscode | User needs to be able to control whether or not the issue report seaches duplicates | Issue Type: <b>Bug</b>
We introduced several settings that allow users to control whether or when VS Code talks to online services. They show when searching for `@tag:usesOnlineServices` in the settings editor.
There is no way to control online service usage for the issue reporter. Neither a checkbox on the issue reporter nor a setting. There should be one since we don't talk to GH directly.
VS Code version: Code - Insiders 1.29.0-insider (4626bc0e1b679d555dd6f034ece35ffc394b3bb7, 2018-10-29T06:11:45.152Z)
OS version: Darwin x64 18.0.0
<!-- generated by issue reporter --> | feature-request,issue-reporter | low | Critical |
375,168,593 | vscode | HTML editing features don't work well in PHP files | <!-- Please search existing issues to avoid creating duplicates. -->
<!-- Also please test using the latest insiders build to make sure your issue has not already been fixed: https://code.visualstudio.com/insiders/ -->
<!-- Use Help > Report Issue to prefill these. -->
- VSCode Version: Version: 1.28.2 (user setup)
Commit: 7f3ce96ff4729c91352ae6def877e59c561f4850
Date: 2018-10-17T00:23:51.859Z
Electron: 2.0.9
Chrome: 61.0.3163.100
Node.js: 8.9.3
V8: 6.1.534.41
Architecture: x64
- OS Version: Windows 7
Steps to Reproduce:
1. Create new PHP file
2. Type in div.class, hit tab, hit enter
Current Solution:
Change file type to HTML, and then back to PHP
Proposed solution:
In a file type where HTML tab-completion works, HTML auto-indenting should also work
<!-- Launch with `code --disable-extensions` to check. -->
Does this issue occur when all extensions are disabled?: Quick Google search did not provide clear instructions on how to perform this in Windows.
| feature-request,php | low | Major |
375,171,684 | flutter | flutter_tool should support discovery of observatory instances without log scanning | Internal: b/124499560
This might involve tmp files for active ports or some other methodology. Currently Android uses log scanning, haven't looked into iOS too much
cc @mehmetf | platform-android,tool,a: existing-apps,customer: dream (g3),P3,team-android,triaged-android | medium | Major |
375,188,824 | rust | Incorrect error note, possibly incorrect error altogether ? | In both stable and the latest nightly, [the following code](https://play.rust-lang.org/?version=nightly&mode=debug&edition=2018&gist=ac030833cb7e72ad043b7a07f48e766a):
```rust
trait Trait {}
impl<T> Trait for Box<T> {}
impl<T: 'static + Copy> Trait for T {}
```
Will fail with this message:
```
error[E0119]: conflicting implementations of trait `Trait` for type `std::boxed::Box<_>`:
--> src/main.rs:3:1
|
2 | impl<T> Trait for Box<T> {}
| ------------------------ first implementation here
3 | impl<T: 'static + Copy> Trait for T {}
| ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ conflicting implementation for `std::boxed::Box<_>`
|
= note: downstream crates may implement trait `std::marker::Copy` for type `std::boxed::Box<_>`
```
If I understand how traits work across crates, the note on that error is false.
While I'm opening an issue about the erroneous note, I'm concerned that the error altogether might be mistaken. Is there a reason for my example code to not be allowed ?
Thanks for your time. | C-enhancement,A-diagnostics,T-compiler | low | Critical |
375,200,061 | TypeScript | JavaScript: default parameter causes type to be incorrectly inferred | <!-- π¨ STOP π¨ π¦π§π’π£ π¨ πΊπ»πΆπ· π¨
Half of all issues filed here are duplicates, answered in the FAQ, or not appropriate for the bug tracker. Even if you think you've found a *bug*, please read the FAQ first, especially the Common "Bugs" That Aren't Bugs section!
Please help us by doing the following steps before logging an issue:
* Search: https://github.com/Microsoft/TypeScript/search?type=Issues
* Read the FAQ: https://github.com/Microsoft/TypeScript/wiki/FAQ
Please fill in the *entire* template below.
-->
From https://github.com/Microsoft/vscode/issues/62013
<!-- Please try to reproduce the issue with `typescript@next`. It may have already been fixed. -->
**TypeScript Version:** 3.2.0-dev.20181027
<!-- Search terms you tried before logging this (so others can find this issue more easily) -->
**Search Terms:**
**Code**
For the javascript:
```js
var SomeObject = {
SomeFunction: function (SomeInput) {
return SomeInput * 2;
},
SomeOtherFunction: function (SomeOtherInput, SomeOptionalInput = SomeObject.SomeFunction(2)) {
return SomeOtherInput * SomeOptionalInput;
}
};
SomeObject
```
Hover over `SomeObject`
**Bug:**
Type is `any`. If the default parameter is removed then the type is correct | Suggestion,Awaiting More Feedback,Domain: JavaScript | low | Critical |
375,214,364 | go | database/sql: Documentation clarification regarding Tx | The docs state:
```
Tx is an in-progress database transaction.
A transaction must end with a call to Commit or Rollback.
After a call to Commit or Rollback, all operations on the transaction fail with ErrTxDone.
The statements prepared for a transaction by calling the transaction's Prepare or Stmt methods are closed by the call to Commit or Rollback.
```
The documentation should clarify if that is only the case if Commit or Rollback return a nil error.
What happens if they return a non-nil error? Should we try and call Commit or Rollback again? Will other operations still return ErrTxDone? | Documentation,help wanted,NeedsFix | low | Critical |
375,222,838 | pytorch | Non-Zero Padding in Convolution Module | ## π Feature
Non-Zero Padding is currently only supported by explicitly padding the input before doing the convolution.
It would be better (more memory efficient, faster and userfrindly) to add an additional parameter "padding_value" to the convolution module.
## Motivation
Zero padding is sufficient to control the dimensions of the output. However, there is no clear motivation for the padding to be zero-valued. On the contrary, zero padding might even deteriorate the end results. Thus, the user should be able to specify an arbitrary value for the padding.
## Pitch
To realise the additional parameter the following changes would needed to be made.
- Python API (Conv1d, Conv2d, Conv3d): [torch/nn/modules/conv.py](https://github.com/pytorch/pytorch/blob/master/torch/nn/modules/conv.py)
- Convolution Logic: [aten/src/ATen/native/Convolution.cpp](https://github.com/pytorch/pytorch/blob/master/aten/src/ATen/native/Convolution.cpp)
- Header Generation: [aten/src/ATen/nn.yaml](https://github.com/pytorch/pytorch/blob/master/aten/src/ATen/nn.yaml), [aten/src/ATen/native/native_functions.yaml](https://github.com/pytorch/pytorch/blob/master/aten/src/ATen/native/native_functions.yaml), [/tools/autograd/derivatives.yaml](https://github.com/pytorch/pytorch/blob/master/tools/autograd/derivatives.yaml)
- THNN, THCUNN headers: [aten/src/THNN/generic/THNN.h](https://github.com/pytorch/pytorch/blob/master/aten/src/THNN/generic/THNN.h) [aten/src/THCUNN/generic/THCUNN.h](https://github.com/pytorch/pytorch/blob/master/aten/src/THCUNN/generic/THCUNN.h)
- Convolution Implementation (in THNN and THCUNN): [SpatialConvolutionMM](https://github.com/pytorch/pytorch/blob/master/aten/src/THNN/generic/SpatialConvolutionMM.c), [SpatialDilatedConvolution](https://github.com/pytorch/pytorch/blob/master/aten/src/THNN/generic/SpatialDilatedConvolution.c)...
- Unfolding operations [im2col](https://github.com/pytorch/pytorch/blob/master/aten/src/THCUNN/im2col.h)
## Additional context
@bengisuelis, @cdemirsoy and I implemented the above discribed modifications for gpu (THCUNN) convolutions and would like to contribute our code.
We did not add the functionality for external librarys (wrapper neeeded) and Cpu executed code (THNN). For those cases we currently throw a runtime error "padding_value not yet supported".
cc @albanD @mruberry @jbschlosser | module: nn,module: convolution,triaged | low | Critical |
375,351,089 | pytorch | [Caffe2]How to accelerate group convolution in Caffe2? | I have update my cuDNN from 6. to 7. but there seems no speedup. The build was forked form the latest source and CUDA=8.0. What should I do to make a further acceleration? | caffe2 | low | Minor |
375,360,487 | pytorch | Use a dill-based multiprocessing library and serialization | ## π Feature
<!-- A clear and concise description of the feature proposal -->
Use a dill-based multiprocessing library to enhance multiprocessing experience.
## Motivation
<!-- Please outline the motivation for the proposal. Is your feature request related to a problem? e.g., I'm always frustrated when [...]. If this is related to another GitHub issue, please link here too -->
Currently multiprocessing uses pickle, which has disadvantages. Not everything can be pickled easily. For example, dynamically created classes and nested classes will fail. If instead of multiprocessing, pytorch used a drop-in replacement that uses dill, e.g. `multiprocess` a fork of multiprocessing, these problems should be alleviated with few or no side-effects.
## Pitch
<!-- A clear and concise description of what you want to happen. -->
Multiprocessing in torch should not be limited by the brittle pickle system. I want to be able to create python programs without the limitations of pickle, but still use pytorch and its multiprocessing system.
## Alternatives
<!-- A clear and concise description of any alternative solutions or features you've considered, if any. -->
## Additional context
<!-- Add any other context or screenshots about the feature request here. -->
| module: multiprocessing,triaged | low | Major |
375,400,928 | create-react-app | Jest CSS enhancement suggestion: don't lose classNames in snapshot tests. | We've been using react-app-rewired with create-react-app v1 and react-scripts-ts for Typescript support for a while, and are starting to look at the new v2.1 release with Typescript support.
One of the enhancements we are using in jest snapshot tests is to output the classnames from css imports in the snapshot. This enables the ability to test parts of a component where a change in props or a user interaction is supposed to result in a change in css being applied to the component (e.g. as a simple make-believe example, changing className from "" to "locallyModified" where there is a `:local(.locallyModified) { color: #FFFF44; }` class in the css).
The snapshot then contains `<div className="locallyModified">` instead of simply `<div>`, which highlights an issue when someone makes a change that breaks the behaviour identifying when the conditional styling should be altered.
The actual cssTransforms.js file is simply:
```javascript
const idObj = new Proxy(
{},
{
get: function getter(target, key) {
return key;
}
}
);
module.exports = idObj;
```
Is it possible to consider adding this to react-scripts/config/jest/cssTransform.js by default? Or is there a reason why it couldn't/shouldn't be widely used? | issue: needs investigation | low | Major |
375,406,835 | pytorch | ASSERT FAILED at /opt/conda/conda-bld/pytorch-nightly_1539602533843/work/aten/src/ATen/core/blob.h:79 | ## π Bug
Hello Great programmers:
When I was using FAIR's platform Detectron to do training with *e2e_mask_rcnn_R-101-FPN_3x_gn.yaml* config file, I faced this issue which indicated me to report one BUG to Pytorch.
<!-- A clear and concise description of what the bug is. -->
```
[I net_dag_utils.cc:102] Operator graph pruning prior to chain compute took: 6.1526e-05 secs
[I net_dag_utils.cc:102] Operator graph pruning prior to chain compute took: 5.3939e-05 secs
[I net_dag_utils.cc:102] Operator graph pruning prior to chain compute took: 7.264e-06 secs
[I net_async_base.h:198] Using specified CPU pool size: 4; NUMA node id: -1
[I net_async_base.h:203] Created new CPU pool, size: 4; NUMA node id: -1
[E net_async_base.cc:422] IsType<T>() ASSERT FAILED at /opt/conda/conda-bld/pytorch-nightly_1539602533843/work/aten/src/ATen/core/blob.h:79, please report a bug to PyTorch. wrong type for the Blob instance. Blob contains nullptr (uninitialized) while caller expects caffe2::Tensor.
Offending Blob name: gpu_0/conv1_gn_s.
Error from operator:
input: "gpu_0/conv1" input: "gpu_0/conv1_gn_s" input: "gpu_0/conv1_gn_b" output: "gpu_0/conv1_gn" output: "gpu_0/conv1_gn_mean" output: "gpu_0/conv1_gn_std" name: "" type: "GroupNorm" arg { name: "use_cudnn" i: 1 } arg { name: "cudnn_exhaustive_search" i: 0 } arg { name: "group" i: 32 } arg { name: "epsilon" f: 1e-05 } device_option { device_type: 1 device_id: 0 } (Get at /opt/conda/conda-bld/pytorch-nightly_1539602533843/work/aten/src/ATen/core/blob.h:79)
frame #0: <unknown function> + 0x277d775 (0x7fa4c1984775 in /home/gengfeng/anaconda3/lib/python3.6/site-packages/caffe2/python/../../torch/lib/libcaffe2_gpu.so)
frame #1: <unknown function> + 0x1321685 (0x7fa4c0528685 in /home/gengfeng/anaconda3/lib/python3.6/site-packages/caffe2/python/../../torch/lib/libcaffe2_gpu.so)
frame #2: caffe2::AsyncNetBase::run(int, int) + 0x16e (0x7fa4ec15f4ee in /home/gengfeng/anaconda3/lib/python3.6/site-packages/caffe2/python/../../torch/lib/libcaffe2.so)
frame #3: <unknown function> + 0x1259972 (0x7fa4ec16e972 in /home/gengfeng/anaconda3/lib/python3.6/site-packages/caffe2/python/../../torch/lib/libcaffe2.so)
frame #4: <unknown function> + 0x124d1cb (0x7fa4ec1621cb in /home/gengfeng/anaconda3/lib/python3.6/site-packages/caffe2/python/../../torch/lib/libcaffe2.so)
frame #5: <unknown function> + 0xafc5c (0x7fa4f6227c5c in /home/gengfeng/anaconda3/bin/../lib/libstdc++.so.6)
frame #6: <unknown function> + 0x76db (0x7fa4fd2806db in /lib/x86_64-linux-gnu/libpthread.so.0)
frame #7: clone + 0x3f (0x7fa4fcfa988f in /lib/x86_64-linux-gnu/libc.so.6)
, op GroupNorm
WARNING workspace.py: 187: Original python traceback for operator `1` in network `generalized_rcnn` in exception above (most recent call last):
WARNING workspace.py: 192: File "tools/train_net.py", line 132, in <module>
WARNING workspace.py: 192: File "tools/train_net.py", line 117, in main
WARNING workspace.py: 192: File "tools/train_net.py", line 127, in test_model
WARNING workspace.py: 192: File "/home/gengfeng/Desktop/projects/DETECTRON/detectron/core/test_engine.py", line 128, in run_inference
WARNING workspace.py: 192: File "/home/gengfeng/Desktop/projects/DETECTRON/detectron/core/test_engine.py", line 108, in result_getter
WARNING workspace.py: 192: File "/home/gengfeng/Desktop/projects/DETECTRON/detectron/core/test_engine.py", line 159, in test_net_on_dataset
WARNING workspace.py: 192: File "/home/gengfeng/Desktop/projects/DETECTRON/detectron/core/test_engine.py", line 235, in test_net
WARNING workspace.py: 192: File "/home/gengfeng/Desktop/projects/DETECTRON/detectron/core/test_engine.py", line 328, in initialize_model_from_cfg
WARNING workspace.py: 192: File "/home/gengfeng/Desktop/projects/DETECTRON/detectron/modeling/model_builder.py", line 124, in create
WARNING workspace.py: 192: File "/home/gengfeng/Desktop/projects/DETECTRON/detectron/modeling/model_builder.py", line 89, in generalized_rcnn
WARNING workspace.py: 192: File "/home/gengfeng/Desktop/projects/DETECTRON/detectron/modeling/model_builder.py", line 229, in build_generic_detection_model
WARNING workspace.py: 192: File "/home/gengfeng/Desktop/projects/DETECTRON/detectron/modeling/optimizer.py", line 54, in build_data_parallel_model
WARNING workspace.py: 192: File "/home/gengfeng/Desktop/projects/DETECTRON/detectron/modeling/model_builder.py", line 169, in _single_gpu_build_func
WARNING workspace.py: 192: File "/home/gengfeng/Desktop/projects/DETECTRON/detectron/modeling/FPN.py", line 63, in add_fpn_ResNet101_conv5_body
WARNING workspace.py: 192: File "/home/gengfeng/Desktop/projects/DETECTRON/detectron/modeling/FPN.py", line 104, in add_fpn_onto_conv_body
WARNING workspace.py: 192: File "/home/gengfeng/Desktop/projects/DETECTRON/detectron/modeling/ResNet.py", line 48, in add_ResNet101_conv5_body
WARNING workspace.py: 192: File "/home/gengfeng/Desktop/projects/DETECTRON/detectron/modeling/ResNet.py", line 99, in add_ResNet_convX_body
WARNING workspace.py: 192: File "/home/gengfeng/Desktop/projects/DETECTRON/detectron/modeling/ResNet.py", line 264, in basic_gn_stem
WARNING workspace.py: 192: File "/home/gengfeng/Desktop/projects/DETECTRON/detectron/modeling/detector.py", line 450, in ConvGN
WARNING workspace.py: 192: File "/home/gengfeng/anaconda3/lib/python3.6/site-packages/caffe2/python/cnn.py", line 165, in SpatialGN
WARNING workspace.py: 192: File "/home/gengfeng/anaconda3/lib/python3.6/site-packages/caffe2/python/brew.py", line 107, in scope_wrapper
WARNING workspace.py: 192: File "/home/gengfeng/anaconda3/lib/python3.6/site-packages/caffe2/python/helpers/normalization.py", line 206, in spatial_gn
Traceback (most recent call last):
File "tools/train_net.py", line 132, in <module>
main()
File "tools/train_net.py", line 117, in main
test_model(checkpoints['final'], args.multi_gpu_testing, args.opts)
File "tools/train_net.py", line 127, in test_model
check_expected_results=True,
File "/home/gengfeng/Desktop/projects/DETECTRON/detectron/core/test_engine.py", line 128, in run_inference
all_results = result_getter()
File "/home/gengfeng/Desktop/projects/DETECTRON/detectron/core/test_engine.py", line 108, in result_getter
multi_gpu=multi_gpu_testing
File "/home/gengfeng/Desktop/projects/DETECTRON/detectron/core/test_engine.py", line 159, in test_net_on_dataset
weights_file, dataset_name, proposal_file, output_dir, gpu_id=gpu_id
File "/home/gengfeng/Desktop/projects/DETECTRON/detectron/core/test_engine.py", line 258, in test_net
model, im, box_proposals, timers
File "/home/gengfeng/Desktop/projects/DETECTRON/detectron/core/test.py", line 66, in im_detect_all
model, im, cfg.TEST.SCALE, cfg.TEST.MAX_SIZE, boxes=box_proposals
File "/home/gengfeng/Desktop/projects/DETECTRON/detectron/core/test.py", line 158, in im_detect_bbox
workspace.RunNet(model.net.Proto().name)
File "/home/gengfeng/anaconda3/lib/python3.6/site-packages/caffe2/python/workspace.py", line 219, in RunNet
StringifyNetName(name), num_iter, allow_fail,
File "/home/gengfeng/anaconda3/lib/python3.6/site-packages/caffe2/python/workspace.py", line 180, in CallWithExceptionIntercept
return func(*args, **kwargs)
RuntimeError: IsType<T>() ASSERT FAILED at /opt/conda/conda-bld/pytorch-nightly_1539602533843/work/aten/src/ATen/core/blob.h:79, please report a bug to PyTorch. wrong type for the Blob instance. Blob contains nullptr (uninitialized) while caller expects caffe2::Tensor.
Offending Blob name: gpu_0/conv1_gn_s.
Error from operator:
input: "gpu_0/conv1" input: "gpu_0/conv1_gn_s" input: "gpu_0/conv1_gn_b" output: "gpu_0/conv1_gn" output: "gpu_0/conv1_gn_mean" output: "gpu_0/conv1_gn_std" name: "" type: "GroupNorm" arg { name: "use_cudnn" i: 1 } arg { name: "cudnn_exhaustive_search" i: 0 } arg { name: "group" i: 32 } arg { name: "epsilon" f: 1e-05 } device_option { device_type: 1 device_id: 0 } (Get at /opt/conda/conda-bld/pytorch-nightly_1539602533843/work/aten/src/ATen/core/blob.h:79)
frame #0: <unknown function> + 0x277d775 (0x7fa4c1984775 in /home/gengfeng/anaconda3/lib/python3.6/site-packages/caffe2/python/../../torch/lib/libcaffe2_gpu.so)
frame #1: <unknown function> + 0x1321685 (0x7fa4c0528685 in /home/gengfeng/anaconda3/lib/python3.6/site-packages/caffe2/python/../../torch/lib/libcaffe2_gpu.so)
frame #2: caffe2::AsyncNetBase::run(int, int) + 0x16e (0x7fa4ec15f4ee in /home/gengfeng/anaconda3/lib/python3.6/site-packages/caffe2/python/../../torch/lib/libcaffe2.so)
frame #3: <unknown function> + 0x1259972 (0x7fa4ec16e972 in /home/gengfeng/anaconda3/lib/python3.6/site-packages/caffe2/python/../../torch/lib/libcaffe2.so)
frame #4: <unknown function> + 0x124d1cb (0x7fa4ec1621cb in /home/gengfeng/anaconda3/lib/python3.6/site-packages/caffe2/python/../../torch/lib/libcaffe2.so)
frame #5: <unknown function> + 0xafc5c (0x7fa4f6227c5c in /home/gengfeng/anaconda3/bin/../lib/libstdc++.so.6)
frame #6: <unknown function> + 0x76db (0x7fa4fd2806db in /lib/x86_64-linux-gnu/libpthread.so.0)
frame #7: clone + 0x3f (0x7fa4fcfa988f in /lib/x86_64-linux-gnu/libc.so.6)
```
## To Reproduce
Steps to reproduce the behavior:
1.
1.
1.
<!-- If you have a code sample, error messages, stack traces, please provide it here as well -->
## Expected behavior
no exception or bug for training and testing.
<!-- A clear and concise description of what you expected to happen. -->
## Environment
Please copy and paste the output from our
[environment collection script](https://raw.githubusercontent.com/pytorch/pytorch/master/torch/utils/collect_env.py)
(or fill out the checklist below manually).
You can get the script and run it with:
```
wget https://raw.githubusercontent.com/pytorch/pytorch/master/torch/utils/collect_env.py
# For security purposes, please check the contents of collect_env.py before running it.
python collect_env.py
```
gengfeng@ai-work-4:~/Downloads$ python collect_env.py
Collecting environment information...
PyTorch version: 1.0.0.dev20181015
Is debug build: No
CUDA used to build PyTorch: 9.0.176
OS: Ubuntu 18.04.1 LTS
GCC version: (Ubuntu 5.5.0-12ubuntu1) 5.5.0 20171010
CMake version: version 3.11.4
Python version: 3.6
Is CUDA available: Yes
CUDA runtime version: 9.0.176
GPU models and configuration: GPU 0: GeForce GTX 1080
Nvidia driver version: 390.87
cuDNN version: Probably one of the following:
/usr/local/cuda-9.0/lib64/libcudnn.so
/usr/local/cuda-9.0/lib64/libcudnn.so.7
/usr/local/cuda-9.0/lib64/libcudnn.so.7.2.1
/usr/local/cuda-9.0/lib64/libcudnn_static.a
/usr/local/cuda-9.1/lib64/libcudnn.so
/usr/local/cuda-9.1/lib64/libcudnn.so.7.1.3
/usr/local/cuda-9.1/lib64/libcudnn_static.a
Versions of relevant libraries:
[pip] Could not collect
[conda] cuda91 1.0 h4c16780_0 pytorch
[conda] pytorch-nightly 1.0.0.dev20181015 py3.6_cuda9.0.176_cudnn7.1.2_0 pytorch
[conda] torch 0.4.0 <pip>
[conda] torchvision 0.2.1 <pip>
```
My own configs *e2e_mask_rcnn_R-101-FPN_3x_gn.yaml*
MODEL:
TYPE: generalized_rcnn
CONV_BODY: FPN.add_fpn_ResNet101_conv5_body
NUM_CLASSES: 2
FASTER_RCNN: True
MASK_ON: True
NUM_GPUS: 1
SOLVER:
WEIGHT_DECAY: 0.0001
LR_POLICY: steps_with_decay
BASE_LR: 0.002
GAMMA: 0.1
MAX_ITER: 70000
STEPS: [0, 40000, 60000]
FPN:
FPN_ON: True
MULTILEVEL_ROIS: True
MULTILEVEL_RPN: True
USE_GN: True # Note: use GN on the FPN-specific layers
RESNETS:
STRIDE_1X1: False # default True for MSRA; False for C2 or Torch models
TRANS_FUNC: bottleneck_gn_transformation # Note: this is a GN bottleneck transform
STEM_FUNC: basic_gn_stem # Note: this is a GN stem
SHORTCUT_FUNC: basic_gn_shortcut # Note: this is a GN shortcut
FAST_RCNN:
ROI_BOX_HEAD: fast_rcnn_heads.add_roi_Xconv1fc_gn_head # Note: this is a Conv GN head
ROI_XFORM_METHOD: RoIAlign
ROI_XFORM_RESOLUTION: 7
ROI_XFORM_SAMPLING_RATIO: 2
MRCNN:
ROI_MASK_HEAD: mask_rcnn_heads.mask_rcnn_fcn_head_v1up4convs_gn # Note: this is a GN mask head
RESOLUTION: 28 # (output mask resolution) default 14
ROI_XFORM_METHOD: RoIAlign
ROI_XFORM_RESOLUTION: 14 # default 7
ROI_XFORM_SAMPLING_RATIO: 2 # default 0
DILATION: 1 # default 2
CONV_INIT: MSRAFill # default GaussianFill
TRAIN:
WEIGHTS: https://s3-us-west-2.amazonaws.com/detectron/ImageNetPretrained/47592356/R-101-GN.pkl # Note: a GN pre-trained model
DATASETS: ('coco_labelme_train',)
SCALES: (700,)
MAX_SIZE: 1333
BATCH_SIZE_PER_IM: 64
RPN_PRE_NMS_TOP_N: 2000 # Per FPN level
TEST:
DATASETS: ('coco_labelme_val',)
SCALE: 700
MAX_SIZE: 1333
NMS: 0.5
RPN_PRE_NMS_TOP_N: 1000 # Per FPN level
RPN_POST_NMS_TOP_N: 1000
OUTPUT_DIR: .
```
## Additional context
<!-- Add any other context about the problem here. -->
By the way, *e2e_mask_rcnn_R-50-FPN_1x.yaml* config works fine for me.
Waiting your response, thank you . | caffe2 | low | Critical |
375,451,642 | go | text/template: confusing overlay template behavior when it starts with a BOM | The program below prints `Master: overlay` once, the second prints nothing. And no error. The program is a variation of this example: https://golang.org/pkg/html/template/#example_Template_block -- which is how we do template "inheritance" in Hugo. And judging by the issue reports in this area, adding a BOM marker seems to be a fairly common practice among text editors. I can work around this on my end, but this behavior is very surprising.
https://play.golang.org/p/MQRb5HUk6eH
```go
package main
import (
"fmt"
"log"
"os"
"text/template"
)
func main() {
for _, prefix := range []string{"", "\ufeff"} {
var (
master = `Master: {{block "list" .}}block{{end}}`
overlay = prefix + `{{define "list"}}overlay{{end}}`
)
masterTmpl, err := template.New("master").Parse(master)
if err != nil {
log.Fatal(err)
}
overlayTmpl, err := template.Must(masterTmpl.Clone()).Parse(overlay)
if err != nil {
log.Fatal(err)
}
if err := overlayTmpl.Execute(os.Stdout, ""); err != nil {
log.Fatal(err)
}
fmt.Println()
}
}
```
https://github.com/gohugoio/hugo/issues/4895 | NeedsDecision | low | Critical |
375,455,112 | svelte | Transitions in custom Elements | Seems like there is a problem with svelte-transitions in customElements, js works properly, adding css: animation, right before destroy, but there are no visual effects...
Seems like it can`t set animation for Shadow CSS. | bug,custom element | low | Major |
375,472,847 | create-react-app | Type check test files | ## Bug report
I created a new project with the `--typescript` flag, and added a type for my component's props like so:
```tsx
type AppProps = {
name: string
};
class App extends Component<AppProps> {
render() {
return (
...
);
}
}
```
In index.tsx when I write something like (note the missing prop):
```tsx
ReactDOM.render(<App />, document.getElementById('root')); // name prop is missing
```
I get an error in both my editor (VSCode) and on my console when I run `yarn start`.
However in my tests if I have something like:
```tsx
it('renders without crashing', () => {
const div = document.createElement('div');
ReactDOM.render(<App />, div);
ReactDOM.unmountComponentAtNode(div);
});
```
I get the type error in my editor but **NOT** when I run `yarn test`, the tests run successfully in fact.
Is this intended?
| tag: breaking change,tag: enhancement,issue: typescript | medium | Critical |
375,529,193 | angular | Avoid anchorScrolling DOMException error with hash fragments that are not valid selectors | <!--
PLEASE HELP US PROCESS GITHUB ISSUES FASTER BY PROVIDING THE FOLLOWING INFORMATION.
ISSUES MISSING IMPORTANT INFORMATION MAY BE CLOSED WITHOUT INVESTIGATION.
-->
## I'm submitting a...
<!-- Check one of the following options with "x" -->
<pre><code>
[ ] Regression (a behavior that used to work and stopped working in a new release)
[ ] Bug report <!-- Please search GitHub for a similar issue or PR before submitting -->
[ ] Performance issue
[x] Feature request
[ ] Documentation issue or request
[ ] Support request => Please do not submit support request here, instead see https://github.com/angular/angular/blob/master/CONTRIBUTING.md#question
[ ] Other... Please describe:
</code></pre>
## Current behavior
<!-- Describe how the issue manifests. -->
If `anchorScrolling` is enabled on the Angular router and the app loads a route that has a hash that does not follow an appropriate format expected for a selector, then a `DOMException` error is thrown.
For example, `#somehash` will not return the error, since this is a valid format for an element selector, even if it is not present on the page (scrolling will fail silently in this case). However, `#somedata=other` will return the error, since this is not a valid element selector and `querySelector` doesn't know what to do with it.
## Expected behavior / Feature request
<!-- Describe what the desired behavior would be. -->
I would expect some way to enable using hash fragments that are not valid DOM selectors without an error, possibly by setting an additional configuration option in `ExtraOptions` for the Router (which can be used in conjunction with `anchorScrolling: 'enabled'`). If the error is still present by default, that is fine as it encourages good practices. However, there is a major use case in which avoiding the error is not currently possible if `anchorScrolling` is enabled (detailed below in motivation). Adding a setting to allow the error to not be thrown might be a good option: possibly something like `allowDataHash`.
Alternatively, if there was a way to _exempt_ an individual route from `anchorScrolling` that was set on the module level, that would also be effective in this scenario.
## Minimal reproduction of the problem with instructions
<!--
For bug reports please provide the *STEPS TO REPRODUCE* and if possible a *MINIMAL DEMO* of the problem via
https://stackblitz.com or similar (you can use this template as a starting point: https://stackblitz.com/fork/angular-gitter).
-->
[GitHub repo with instructions and sample link](https://github.com/kmaida/anchorScrolling-fr)
In any Angular 7 application with routing, enable `anchorScrolling` in the RouterModule's `ExtraOptions`:
```
@NgModule({
imports: [RouterModule.forRoot(routes, { anchorScrolling: 'enabled'} )],
exports: [RouterModule]
})
```
Then attempt to access any route with a hash fragment that is not a valid DOM selector. This could be something as simple as:
```
#somehash=data
```
or something more realistic, such as:
```
#access_token=eyJhbGciOiJSUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkZha2UgVG9rZW4iLCJpYXQiOjE1MTYyMzkwMjJ9.fg-Dm3WJDDPt3J18KcIipEka0n92sFbIF9N7JqCLnQ_194uScpG7nt9JOEDHE0lqgmNTKf1xr31X5rQPO1HkmGMPzQQ64TLoyr1qs8azzdpJKdm3w4R5qn9Z52zA1deIa1-dBShXrC0ynhXBCXaWaCymRD7n6duyBbuZAmAieaI&expires_in=86400
```
## What is the motivation / use case for changing the behavior?
<!-- Describe the motivation or the concrete use case. -->
There is a **major use case** for data being passed in a hash fragment, and it is done this way _in accordance with IETF standards_:
When conforming to [OIDC implicit grant standards](https://tools.ietf.org/html/rfc6749#section-4.2) for authentication in Single Page Applications, data is returned from any OIDC-conformant authorization server (including but not limited to Auth0, Okta, Gigya, Ping Identity, Cognito, etc.) in a hash fragment. This hash fragment follows a format that resembles the following:
```
#access_token=<jwt token>&expires_in=86400
```
The most common way to handle this in SPAs is to set up a callback route. This is the route that the authorization server redirects back to (`redirect_uri`) and adds the hash data to. In the application, the hash is parsed and then removed and the user is redirected within the SPA.
If no authorization server is being used, throwing the error may normally be fine, as it is likely expected that if a user is passing data in the URL, they should use query parameters with `?` instead of a hash fragment with `#`.
However, it is currently not possible to avoid this error while using implicit grant with OIDC-conformant authorization servers using redirect mode with `anchorScrolling` enabled. The primary current workaround is to remove `anchorScrolling` and re-implement anchor scrolling functionality _manually_ instead.
## Environment
<pre><code>
Angular version: 7.0.1
<!-- Check whether this is still an issue in the most recent Angular version -->
</code></pre>
| type: bug/fix,freq3: high,area: router,state: confirmed,P3 | low | Critical |
375,573,600 | go | x/build/cmd/gomote: 'gomote ssh' on plan9 can't see files installed by 'gomote put' | ### What did you do?
```
~$ gomote create plan9-386
user-bcmills-plan9-386-0
~$ gomote put14 user-bcmills-plan9-386-0
~$ gomote push user-bcmills-plan9-386-0
# [β¦]
~/go/src$ gomote run user-bcmills-plan9-386-0 /bin/pwd
/tmp/workdir
~/go/src$ gomote run user-bcmills-plan9-386-0 /bin/ls /tmp/workdir
/tmp/workdir/go
/tmp/workdir/go1.4
~$ gomote ssh user-bcmills-plan9-386-0
$ ssh -p 2222 [email protected] # auth using https://github.com/bcmills.keys
# [β¦]
# `gomote push` and the builders use:
# - workdir: /tmp/workdir
# - GOROOT: /tmp/workdir/go
# - GOPATH: /tmp/workdir/gopath
# - env: GO_BUILDER_NAME=plan9-386 GO_TEST_TIMEOUT_SCALE=2
# Happy debugging.
# [β¦]
cpu% ls /tmp/workdir
ls /tmp/workdir
ls: /tmp/workdir: '/tmp/workdir' file does not exist
cpu%
```
### What did you expect to see?
The files reported by `gomote run [β¦] /bin/ls /tmp/workdir` are also visible when running `ls /tmp/workdir` under `gomote ssh`.
### What did you see instead?
The files placed by `gomote put14` and `gomote push` are not visible to the `gomote ssh` process.
@bradfitz tells me that the the ssh and buildlet processes don't share that part of the filesystem namespace.
@0intro, any ideas? | help wanted,OS-Plan9,Builders,NeedsInvestigation | low | Critical |
375,577,992 | rust | stack overflow with many unused variables | I had a `main()` that was filled with ~5400 `let x = 0;`s, one per line.
When trying to build the program, rustc crashes.
````
warning: unused variable: `x`
--> src/main.rs:5406:6
|
5406 | let x = 0;
| ^ help: consider using `_x` instead
warning: unused variable: `x`
--> src/main.rs:5407:6
|
5407 | let x = 0;
| ^ help: consider using `_x` instead
thread '<unknown>' has overflowed its stack
fatal runtime error: stack overflow
Compile terminated by signal 6
error: Could not compile `many_warn`.
Caused by:
process didn't exit successfully: `/home/matthias/.cargo/bin/sccache rustc --crate-name many_warn src/main.rs --color always --crate-type bin --emit=dep-info,link -C debuginfo=2 -C metadata=1383ca9b11585a98 -C extra-filename=-1383ca9b11585a98 --out-dir /tmp/many_warn/target/debug/deps -C incremental=/tmp/many_warn/target/debug/incremental -L dependency=/tmp/many_warn/target/debug/deps -C target-cpu=native` (exit code: 2)
````
crashes nightly 1.32, beta 1.31.0-beta.1 and stable 1.30.0.
`cargo check` works though.
| I-crash,A-lints,E-needs-test,T-compiler,C-bug | low | Critical |
375,605,199 | flutter | Move diagnostics to separate top level library | The diagnostics functionality as mentioned in issues such as #9568 is too useful to be bundled inside Flutter and tied up with other items that pull in UI-dependencies. The use case and benefit would be developing similar plugins for Dart apps running in non-UI VMs to inspect data structures. | c: new feature,framework,would be a good package,P3,team-framework,triaged-framework | low | Minor |
375,675,061 | TypeScript | Describe the type of missing property in error message | ## Search Terms
- describe missing property
- missing property type
## Suggestion
**In short: When describing a missing type, also describe the shape of the type that is expected, and/or where the interface/type can be located.**
It can be difficult to manually find the type, especially with deep interface nesting and union types. An example of this being difficult is below.
## Use Cases
Basically any time you interact with complicated enough typings, libraries tend to use *a lot* of inheritance and union types. The better the error messages, the more seamless the developer experience is.
## Examples
Real life example of an error message (some interfaces have been abbreviated).
```
src/components/cvr-lookup-field.tsx(24,26): error TS2352: Type 'Props & { children?: ReactNode; }' cannot be converted to type 'TextFieldProps'.
Property 'style' is missing in type 'Props & { children?: ReactNode; }'.
```
So I need `style` - not sure what it is. Let's dig into `TextFieldProps`
```
export interface TextFieldProps extends FieldProps, Omit<MuiTextFieldProps, 'error' | 'name' | 'onChange' | 'value'> {
}
```
Alright, so two interfaces, one with some properties omitted. `FieldProp` is reasonably simple, and I can tell `style` isn't defined there. Let's dig into MuiTextFieldProps
```
export interface StandardTextFieldProps extends BaseTextFieldProps {
variant?: 'standard';
}
export interface OutlinedTextFieldProps extends BaseTextFieldProps {
variant: 'outlined';
}
export type MuiTextFieldProps = StandardTextFieldProps | OutlinedTextFieldProps;
```
Let's go deeper..
```
export interface BaseTextFieldProps
extends StandardProps<FormControlProps, TextFieldClassKey, 'onChange' | 'defaultValue'> {
//...
}
```
And at some point you give up, because these hierarchies can get *very* deep. I wanted to find `style` to prove a point for this issue, but I was unable to.
This to me demonstrates how problematic this is - even spending a pretty long time searching for this attribute to file an issue, I was unable to find it.
## Error Message Suggestions
Change the error message from:
```
src/components/cvr-lookup-field.tsx(24,26): error TS2352: Type 'Props & { children?: ReactNode; }' cannot be converted to type 'TextFieldProps'.
Property 'style' is missing in type 'Props & { children?: ReactNode; }'.
```
To this (describing the type)
```
src/components/cvr-lookup-field.tsx(24,26): error TS2352: Type 'Props & { children?: ReactNode; }' cannot be converted to type 'TextFieldProps'.
Property 'style' of type '"red" | "blue" | "green"' is missing in type 'Props & { children?: ReactNode; }'.
```
Or this (describing where to find it):
```
src/components/cvr-lookup-field.tsx(24,26): error TS2352: Type 'Props & { children?: ReactNode; }' cannot be converted to type 'TextFieldProps'.
Property 'style' declared in interface "FooBar" is missing in type 'Props & { children?: ReactNode; }'.
```
## Checklist
My suggestion meets these guidelines:
* [X] This wouldn't be a breaking change in existing TypeScript / JavaScript code
* [X] This wouldn't change the runtime behavior of existing JavaScript code
* [X] This could be implemented without emitting different JS based on the types of the expressions
* [X] This isn't a runtime feature (e.g. new expression-level syntax)
| Suggestion,Committed,Domain: Error Messages | low | Critical |
375,691,975 | TypeScript | "Import assignment cannot be used when targeting ECMAScript modules." should come with a codefix | **TypeScript Version:** 3.2.0-dev.20181030
**Code**
**a.ts**
```ts
import abs = require("abs");
```
**tsconfig.json**
```ts
{
"compilerOptions": {
"target": "esnext"
}
}
```
The error message is `Import assignment cannot be used when targeting ECMAScript modules. Consider using 'import * as ns from "mod"', 'import {a} from "mod"', 'import d from "mod"', or another module format instead.`. This indicates that we could provide a code fix.
**Expected behavior:**
There is a codefix that offers to change this to `import abs from "abs";`, or `import * as abs from "abs";`. If `--esModuleInterop` is set and the imported value is not used only as a namespace, we should prefer a default import.
**Actual behavior:**
No codefix. | Suggestion,In Discussion,Domain: Quick Fixes | low | Critical |
375,733,745 | flutter | Provide API in TextField to open the keyboard | From https://github.com/flutter/flutter/issues/15882#issuecomment-433563683:
I've just discovered calling `FocusScope.of(context).requestFocus(...)` is not good enough. It does not [request for the keyboard](https://github.com/flutter/flutter/blob/9bf8502fef3f35d8d3ba692ce0383e9f55a34624/packages/flutter/lib/src/material/text_field.dart#L491). This can be an issue in the following case:
1. Tap on text field. We provide it focus. This opens the keyboard.
2. Tap Android back button. Keyboard is dismissed. Field still has focus.
3. Tap on text field again. We try to provide it focus again but this doesn't do anything. **There is no way to get the keyboard back up.**
In order to display the keyboard, we need to call `requestKeyboard` on the `EditableTextState` but this is [not exposed to us](https://github.com/flutter/flutter/blob/9bf8502fef3f35d8d3ba692ce0383e9f55a34624/packages/flutter/lib/src/material/text_field.dart#L441) as the global key is private. | a: text input,c: new feature,framework,engine,P2,team-engine,triaged-engine | low | Critical |
375,782,215 | go | runtime/race: windows race out of memory allocating heap arena map | https://build.golang.org/log/48dc600dd822d082a10c753b50f3123d8cb2e86d
```
...
FAIL runtime/race 46.500s
==996==ERROR: ThreadSanitizer failed to allocate 0x000004000000 (67108864) bytes at 0x04008c000000 (error code: 1455)
runtime: newstack sp=0x588fe50 stack=[0xc002168000, 0xc00216c000]
morebuf={pc:0x407319 sp:0x588fe60 lr:0x0}
sched={pc:0x474370 sp:0x588fe58 lr:0x0 ctxt:0x0}
runtime: gp=0xc000026c00, goid=27, gp->status=0x2
runtime: split stack overflow: 0x588fe50 < 0xc002168000
fatal error: runtime: split stack overflow
runtime stack:
runtime.throw(0x67ddba, 0x1d)
C:/workdir/go/src/runtime/panic.go:608 +0x79
runtime.newstack()
C:/workdir/go/src/runtime/stack.go:995 +0x86f
runtime.morestack()
C:/workdir/go/src/runtime/asm_amd64.s:429 +0x97
goroutine 27 [running]:
runtime.sigpanic()
C:/workdir/go/src/runtime/signal_windows.go:201 +0x170 fp=0x588fe60 sp=0x588fe58 pc=0x474370
created by testing.(*T).Run
C:/workdir/go/src/testing/testing.go:901 +0x686
goroutine 1 [chan receive]:
testing.(*T).Run(0xc0000b6000, 0x679341, 0xf, 0x683df8, 0xc000075c01)
C:/workdir/go/src/testing/testing.go:902 +0x6be
testing.runTests.func1(0xc0000b6000)
C:/workdir/go/src/testing/testing.go:1142 +0xb0
testing.tRunner(0xc0000b6000, 0xc000075dd0)
C:/workdir/go/src/testing/testing.go:850 +0x16a
testing.runTests(0xc000048460, 0x7f6e20, 0x9, 0x9, 0x80)
C:/workdir/go/src/testing/testing.go:1140 +0x4f3
testing.(*M).Run(0xc0000a6000, 0x0)
C:/workdir/go/src/testing/testing.go:1057 +0x2f7
main.main()
_testmain.go:64 +0x229
goroutine 6 [select (no cases)]:
runtime/trace_test.TestTraceSymbolize.func1()
C:/workdir/go/src/runtime/trace/trace_stack_test.go:41 +0x37
created by runtime/trace_test.TestTraceSymbolize
C:/workdir/go/src/runtime/trace/trace_stack_test.go:40 +0x137
goroutine 7 [chan send (nil chan)]:
runtime/trace_test.TestTraceSymbolize.func2()
C:/workdir/go/src/runtime/trace/trace_stack_test.go:45 +0x52
created by runtime/trace_test.TestTraceSymbolize
C:/workdir/go/src/runtime/trace/trace_stack_test.go:43 +0x14f
goroutine 8 [chan receive (nil chan)]:
runtime/trace_test.TestTraceSymbolize.func3()
C:/workdir/go/src/runtime/trace/trace_stack_test.go:49 +0x3e
created by runtime/trace_test.TestTraceSymbolize
C:/workdir/go/src/runtime/trace/trace_stack_test.go:47 +0x167
goroutine 130 [select (no cases)]:
runtime/trace_test.TestTraceStress.func9()
C:/workdir/go/src/runtime/trace/trace_test.go:307 +0x3c
created by runtime/trace_test.TestTraceStress
C:/workdir/go/src/runtime/trace/trace_test.go:305 +0x717
FAIL runtime/trace 51.330s
ok sort 2.255s
ok strconv 8.116s
ok strings 46.369s
--- FAIL: TestMutexMisuse (0.16s)
mutex_test.go:176: Mutex.Unlock: did not find failure with message about unlocked lock: exit status 2
fatal error: out of memory allocating heap arena map
runtime stack:
runtime.throw(0x675b79, 0x27)
C:/workdir/go/src/runtime/panic.go:608 +0x79 fp=0x22fae0 sp=0x22fab0 pc=0x45f499
runtime.(*mheap).sysAlloc(0x7fd740, 0x2000, 0x48, 0x48)
C:/workdir/go/src/runtime/malloc.go:627 +0x4d7 fp=0x22fb70 sp=0x22fae0 pc=0x43c247
runtime.(*mheap).grow(0x7fd740, 0x1, 0x0)
C:/workdir/go/src/runtime/mheap.go:942 +0x49 fp=0x22fbc8 sp=0x22fb70 pc=0x454c09
runtime.(*mheap).allocSpanLocked(0x7fd740, 0x1, 0x816fe8, 0x560010000a7)
C:/workdir/go/src/runtime/mheap.go:866 +0x3c8 fp=0x22fc10 sp=0x22fbc8 pc=0x454ac8
runtime.(*mheap).alloc_m(0x7fd740, 0x1, 0x23, 0x22fd90)
C:/workdir/go/src/runtime/mheap.go:710 +0x100 fp=0x22fc50 sp=0x22fc10 pc=0x454280
runtime.(*mheap).alloc.func1()
C:/workdir/go/src/runtime/mheap.go:779 +0x53 fp=0x22fc88 sp=0x22fc50 pc=0x489db3
runtime.(*mheap).alloc(0x7fd740, 0x1, 0x10023, 0x22fd10)
C:/workdir/go/src/runtime/mheap.go:778 +0x91 fp=0x22fcd8 sp=0x22fc88 pc=0x4544d1
runtime.(*mcentral).grow(0x7fe540, 0x0)
C:/workdir/go/src/runtime/mcentral.go:256 +0x9a fp=0x22fd20 sp=0x22fcd8 pc=0x447eba
runtime.(*mcentral).cacheSpan(0x7fe540, 0x2a60000)
C:/workdir/go/src/runtime/mcentral.go:106 +0x2b4 fp=0x22fd68 sp=0x22fd20 pc=0x4479d4
runtime.(*mcache).refill(0x2a60000, 0x23)
C:/workdir/go/src/runtime/mcache.go:135 +0x8d fp=0x22fd88 sp=0x22fd68 pc=0x44749d
runtime.(*mcache).nextFree(0x2a60000, 0x489223, 0x4000, 0x0, 0x817040)
C:/workdir/go/src/runtime/malloc.go:749 +0x8f fp=0x22fdc0 sp=0x22fd88 pc=0x43c3df
runtime.mallocgc(0x100, 0x63de60, 0x2a60001, 0x2a60000)
C:/workdir/go/src/runtime/malloc.go:902 +0x7b8 fp=0x22fe60 sp=0x22fdc0 pc=0x43cd48
runtime.newobject(0x63de60, 0x7f94a0)
C:/workdir/go/src/runtime/malloc.go:1031 +0x3f fp=0x22fe90 sp=0x22fe60 pc=0x43d15f
runtime.mcommoninit(0x7f94a0)
C:/workdir/go/src/runtime/proc.go:635 +0x126 fp=0x22fec8 sp=0x22fe90 pc=0x462216
runtime.schedinit()
C:/workdir/go/src/runtime/proc.go:541 +0xb8 fp=0x22ff30 sp=0x22fec8 pc=0x461ea8
runtime.rt0_go(0x22ff60, 0x773f59cd, 0x22ff60, 0x0, 0x773f59cd, 0x0, 0x0, 0x0, 0x0, 0x0, ...)
C:/workdir/go/src/runtime/asm_amd64.s:195 +0x126 fp=0x22ff38 sp=0x22ff30 pc=0x48bc86
...
``` | OS-Windows,NeedsInvestigation,compiler/runtime | low | Critical |
375,810,518 | pytorch | cannot run mobilenet_v2_quantized on pytorch/caffe2 | ## β Questions and Help
I am trying to run mobilenet_v2_quantized (https://github.com/caffe2/models/tree/master/mobilenet_v2_quantized) on pytorch/caffe2 repo which supports int8 .
after compiling pytorch on tx2 and run the model, I meet the following error:
```
WARNING:root:This caffe2 python run does not have GPU support. Will run in CPU only mode.
WARNING:caffe2.python.workspace:Original python traceback for operator `-2110861432` in network `mobilenet_v2_quant` in exception above (most recent call last):
Traceback (most recent call last):
File "test_caffe2.py", line 470, in <module>
net_def = createNet(pred_net)
File "test_caffe2.py", line 406, in createNet
workspace.CreateNet(net_def, overwrite=True)
File "/home/gg/pytorch/build/caffe2/python/workspace.py", line 154, in CreateNet
StringifyProto(net), overwrite,
File "/home/gg/pytorch/build/caffe2/python/workspace.py", line 180, in CallWithExceptionIntercept
return func(*args, **kwargs)
RuntimeError: [enforce fail at operator.cc:46] blob != nullptr. op NCHW2NHWC: Encountered a non-existing input blob: data
frame #0: c10::ThrowEnforceNotMet(char const*, int, char const*, std::__cxx11::basic_string<char, std::char_traits<char>, std::allocator<char> > const&, void const*) + 0x7c (0x7f5ac89ed4 in /home/gg/pytorch/build/lib/libc10.so)
frame #1: caffe2::OperatorBase::OperatorBase(caffe2::OperatorDef const&, caffe2::Workspace*) + 0x560 (0x7f5bc5fa18 in /home/gg/pytorch/build/lib/libcaffe2.so)
frame #2: <unknown function> + 0x13e5efc (0x7f5c088efc in /home/gg/pytorch/build/lib/libcaffe2.so)
frame #3: std::_Function_handler<std::unique_ptr<caffe2::OperatorBase, std::default_delete<caffe2::OperatorBase> > (caffe2::OperatorDef const&, caffe2::Workspace*), std::unique_ptr<caffe2::OperatorBase, std::default_delete<caffe2::OperatorBase> > (*)(caffe2::OperatorDef const&, caffe2::Workspace*)>::_M_invoke(std::_Any_data const&, caffe2::OperatorDef const&, caffe2::Workspace*&&) + 0x34 (0x7f5cb5dd9c in /home/gg/pytorch/build/caffe2/python/caffe2_pybind11_state.cpython-35m-aarch64-linux-gnu.so)
frame #4: <unknown function> + 0xfbacfc (0x7f5bc5dcfc in /home/gg/pytorch/build/lib/libcaffe2.so)
frame #5: <unknown function> + 0xfbcd0c (0x7f5bc5fd0c in /home/gg/pytorch/build/lib/libcaffe2.so)
frame #6: caffe2::CreateOperator(caffe2::OperatorDef const&, caffe2::Workspace*, int) + 0x430 (0x7f5bc60898 in /home/gg/pytorch/build/lib/libcaffe2.so)
frame #7: caffe2::SimpleNet::SimpleNet(std::shared_ptr<caffe2::NetDef const> const&, caffe2::Workspace*) + 0x3dc (0x7f5bcc016c in /home/gg/pytorch/build/lib/libcaffe2.so)
frame #8: <unknown function> + 0x101eb3c (0x7f5bcc1b3c in /home/gg/pytorch/build/lib/libcaffe2.so)
frame #9: <unknown function> + 0xfa5904 (0x7f5bc48904 in /home/gg/pytorch/build/lib/libcaffe2.so)
frame #10: caffe2::CreateNet(std::shared_ptr<caffe2::NetDef const> const&, caffe2::Workspace*) + 0x90c (0x7f5bc98f94 in /home/gg/pytorch/build/lib/libcaffe2.so)
frame #11: caffe2::Workspace::CreateNet(std::shared_ptr<caffe2::NetDef const> const&, bool) + 0x1e4 (0x7f5bcab824 in /home/gg/pytorch/build/lib/libcaffe2.so)
frame #12: caffe2::Workspace::CreateNet(caffe2::NetDef const&, bool) + 0xa4 (0x7f5bcaca7c in /home/gg/pytorch/build/lib/libcaffe2.so)
frame #13: <unknown function> + 0x51060 (0x7f5cb55060 in /home/gg/pytorch/build/caffe2/python/caffe2_pybind11_state.cpython-35m-aarch64-linux-gnu.so)
frame #14: <unknown function> + 0x512d0 (0x7f5cb552d0 in /home/gg/pytorch/build/caffe2/python/caffe2_pybind11_state.cpython-35m-aarch64-linux-gnu.so)
frame #15: <unknown function> + 0x8edfc (0x7f5cb92dfc in /home/gg/pytorch/build/caffe2/python/caffe2_pybind11_state.cpython-35m-aarch64-linux-gnu.so)
<omitting python frames>
```
the model is running on CPU only
what is the problem?
| caffe2 | low | Critical |
375,818,181 | flutter | Date Time Picker needs seconds picker | it will be a great if you a the native widget Date and Time picker allows seconds to be picked.
Hope it will be in upcoming release. Meanwhile I am trying to add a number picker on after saving the date time.
| c: new feature,framework,f: material design,f: date/time picker,P3,team-design,triaged-design | medium | Major |
375,819,592 | create-react-app | Add baseUrl and paths in tsconfig.json and jsconfig.json | `tsconfig.json` or `jsconfig.json`:
```js
{
"compilerOptions": {
"baseUrl": ".",
"paths": {
"@/*":["src/*"]
}
}
}
```
see https://github.com/facebook/create-react-app/issues/5118
This will help VSCode and other IDE to resolve path in TypeScript & JavaScript. | issue: proposal | high | Critical |
375,831,243 | godot | Viewport Texture generate mipmaps ignored | ___
***Note:** This issue has been confirmed several times already. No need to confirm it further.*
___
Not sure if that's expected behavior but Im using the viewport texture on sprite and I tried to use textureLod(...) so I could get blurry version of that and it doesn't work. Setting flags in editor doesn't help either.
Any workarounds to force generating Lods from Viewport Texture? something like in SCREEN_TEXTURE that has Lods | topic:rendering,confirmed,documentation | medium | Critical |
375,854,448 | youtube-dl | Site support request dimsum.my | - [X] I've **verified** and **I assure** that I'm running youtube-dl **2018.10.29**
### Before submitting an *issue* make sure you have:
- [X] At least skimmed through the [README](https://github.com/rg3/youtube-dl/blob/master/README.md), **most notably** the [FAQ](https://github.com/rg3/youtube-dl#faq) and [BUGS](https://github.com/rg3/youtube-dl#bugs) sections
- [X] [Searched](https://github.com/rg3/youtube-dl/search?type=Issues) the bugtracker for similar issues including closed ones
- [X] Checked that provided video/audio/playlist URLs (if any) are alive and playable in a browser
### What is the purpose of your *issue*?
- [ ] Bug report (encountered problems with youtube-dl)
- [X] Site support request (request for adding support for a new site)
- [ ] Feature request (request for a new functionality)
- [ ] Question
- [ ] Other
```
youtube-dl.exe https://www.dimsum.my/drama/thailand/series-809?lang=en#! -v
[debug] System config: [] [debug] User config: [] [debug] Custom config: [] [debug] Command-line args: ['https://www.dimsum.my/drama/thailand/series-809?lang=en#!', '-v'] [debug] Encodings: locale cp1252, fs mbcs, out cp437, pref cp1252 [debug] youtube-dl version 2018.10.29 [debug] Python version 3.4.4 (CPython) - Windows-10-10.0.17134 [debug] exe versions: ffmpeg N-91500-g3870ed7ab3 [debug] Proxy map: {} [generic] series-809?lang=en#!: Requesting header WARNING: Falling back on generic information extractor. [generic] series-809?lang=en#!: Downloading webpage [generic] series-809?lang=en#!: Extracting information ERROR: Unsupported URL: https://www.dimsum.my/drama/thailand/series-809?lang=en#! Traceback (most recent call last): File "C:\Users\dst\AppData\Roaming\Build archive\youtube-dl\rg3\tmpadzwnijc\build\youtube_dl\YoutubeDL.py", line 792, in extract_info File "C:\Users\dst\AppData\Roaming\Build archive\youtube-dl\rg3\tmpadzwnijc\build\youtube_dl\extractor\common.py", line 508, in extract File "C:\Users\dst\AppData\Roaming\Build archive\youtube-dl\rg3\tmpadzwnijc\build\youtube_dl\extractor\generic.py", line 3298, in _real_extract youtube_dl.utils.UnsupportedError: Unsupported URL: https://www.dimsum.my/drama/thailand/series-809?lang=en#!
```
### If the purpose of this *issue* is a *site support request* please provide all kinds of example URLs support for which should be included (replace following example URLs by **yours**):
- Single video: cant get the link of single video
- Playlist: https://www.dimsum.my/drama/thailand/series-743?lang=en
site support request for www.dimsum.my a account is needed for it but theres a free 30 day trail for those who want to try | geo-restricted,account-needed | low | Critical |
375,903,812 | create-react-app | yarn pnp doesn't work with typescript | Set up a pnp typescript project
`npx create-react-app pnp --use-pnp --typescript` or `npx create-react-app pnp --typescript --use-pnp` order doesn't matter
You then get
```
yarn add v1.12.1
[1/5] π Resolving packages...
[2/5] π Fetching packages...
[3/5] π Linking dependencies...
[5/5] π Building fresh packages...
success Saved lockfile.
success Saved 8 new dependencies.
info Direct dependencies
ββ @types/[email protected]
ββ @types/[email protected]
ββ @types/[email protected]
ββ @types/[email protected]
ββ [email protected]
ββ [email protected]
ββ [email protected]
ββ [email protected]
info All dependencies
ββ @types/[email protected]
ββ @types/[email protected]
ββ @types/[email protected]
ββ @types/[email protected]
ββ [email protected]
ββ [email protected]
ββ [email protected]
ββ [email protected]
β¨ Done in 14.72s.
We detected TypeScript in your project (src/App.test.tsx) and created a tsconfig.json file for you.
It looks like you're trying to use TypeScript but do not have typescript installed.
Please install typescript by running yarn add typescript.
If you are not trying to use TypeScript, please remove the tsconfig.json file from your package root (and any TypeScript files).
Aborting installation.
node --require /Users/bond/Projects/pnp-test/.pnp.js has failed.
Deleting generated file... package.json
Deleting generated file... yarn.lock
Done.
```
---
Environment
```
System:
OS: macOS 10.14
CPU: x64 Intel(R) Core(TM) i7-4770HQ CPU @ 2.20GHz
Binaries:
Node: 8.12.0 - /usr/local/bin/node
Yarn: 1.12.1 - /usr/local/bin/yarn
npm: 6.4.1 - /usr/local/bin/npm
npmGlobalPackages:
create-react-app: Not Found
``` | issue: bug | medium | Critical |
375,930,068 | vscode | Surface the 'origin and 'presentationHint' attributes of a Source in the Loaded Scripts Explorer | When a Source appears in the CALL STACK panel during debugging, its `presentationHint` modifies the row's appearance, and its `origin` displays on the second line of the hover tip.
Please apply `presentationHint` in the same way on the LOADED SCRIPTS panel.
Please also surface `origin` there, either by appending in italics (as currently happens in the OPEN EDITORS panel) or as a second line of the hover tip.
| feature-request,debug | low | Critical |
375,941,545 | rust | "does not live long enough" when returning Option<impl trait> | This doesn't compile:
```
use std::cell::RefCell;
struct B;
struct A {
b: B,
}
impl A {
fn b(&self) -> Option<impl Iterator<Item = &B>> {
Some(::std::iter::once(&self.b))
}
}
fn func(a: RefCell<A>) {
let lock = a.borrow_mut();
if let Some(_b) = lock.b() {}
}
```
However, it compiles when impl trait is replaced with the concrete type:
```
fn b(&self) -> Option<::std::iter::Once<&B>> {
```
It also compiles with impl trait if `Option` is removed:
```
impl A {
fn b(&self) -> impl Iterator<Item = &B> {
::std::iter::once(&self.b)
}
}
fn func(a: RefCell<A>) {
let lock = a.borrow_mut();
let _b = lock.b();
}
``` | A-lifetimes,A-impl-trait,C-bug | low | Minor |
375,970,043 | godot | Tilemap Node : No way to change the offset while painting | <!-- Please search existing issues for potential duplicates before filing yours:
https://github.com/godotengine/godot/issues?q=is%3Aissue
-->
**Godot version:**
<!-- Specify commit hash if non-official. -->
Version : 3.0.6
**OS/device including version:**
<!-- Specify GPU model and drivers if graphics-related. -->
**Issue description:**
<!-- What happened, and what was expected. -->
There is no way to change the offset of tiles while we are painting, so if the tilesets have different dimensions, they can't be connected with each other.
As shown here.
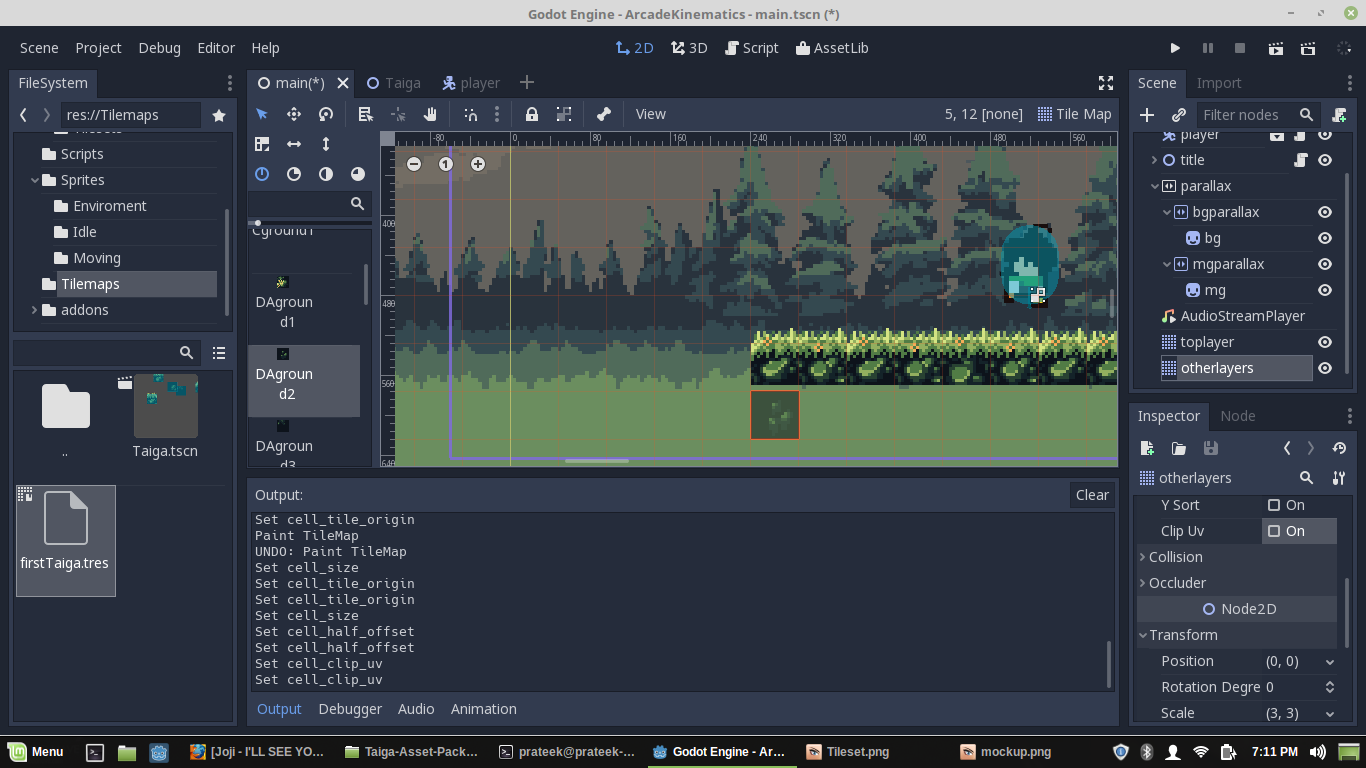
| enhancement,topic:editor | low | Minor |
375,989,194 | go | fmt: docs slightly disorganized and slightly contradictory | Please answer these questions before submitting your issue. Thanks!
### What version of Go are you using (`go version`)?
1.11
### Does this issue reproduce with the latest release?
yes
### What operating system and processor architecture are you using (`go env`)?
N/A
### What did you do?
Read the fmt docs. Someone in IRC asked about padding, and I went and looked, and thought "that seems slightly inaccurate", then reread it and thought "wait that can't be right, can it?" and eventually concluded that things were confusing.
### What did you expect to see?
Something I could follow without much effort.
### What did you see instead?
A confusing tangle. Width and explicit argument indexes are both "immediately preceeding the verb", but actually width won't immediately preceed the verb if precision is present, or an explicit argument index for that matter. I know what width and precision *are* because I'm used to C, but some kind of vague conceptual introduction might be nice. Explicit argument indexes are described separately from every other aspect of the formatting verbs, after an unrelated tangle of things.
Before filing this, I decided to see if I could write something I thought was better. After some effort, I'm pleased to state that I have no idea but I have at least made an effort, so I should have a change submitted for code review "soon". (I think it's justified just by getting the explicit argument indexes moved in with the rest of the stuff.) | Documentation,NeedsFix | low | Minor |
375,997,897 | vscode | Breadcrumb missing on read-only documents supplied by debug adapter | Issue Type: <b>Bug</b>
VS Code version: Code 1.28.2 (7f3ce96ff4729c91352ae6def877e59c561f4850, 2018-10-17T00:23:51.859Z)
OS version: Windows_NT x64 10.0.15063
1. Start debugging something. In my case I am debugging a debug extension written in Typescript, e.g. the mock-debug sample.
2. Use the Pause button to suspend execution at a point where the debug adapter will supply the (read-only) source for display. In my case it was easy to suspend in async_hooks.js.
3. Observe that there's no breadcrumb on the loaded document. Toggling breadcrumb on the View menu has no effect.
4. Set a breakpoint in a source that exists on the local filesystem. Run to that breakpoint and confirm that a breadcrumb appears as expected. Toggling breadcrumb on the View menu works as expected.
Also occurs on current 1.29 Insider. I don't recall if the same problem existed in other breadcrumb-enabled versions (i.e. 1.26+). | feature-request,breadcrumbs | low | Critical |
376,016,817 | svelte | Chrome video autoplay bug when hydrating server-rendered component | If a component contains an autoplaying video, like this:
```html
<video autoplay loop muted>
<source src="https://example.com/video.mp4" type="video/mp4">
</video>
```
and that component is server-rendered and then hydrated in Chrome, the video starts playing for around 500ms until hydration kicks in, then the video pauses.
This only happens with server-rendered components that are hydrated in the browser. Disabling Javascript or server-rendering fixes the issue.
If I add the line
```js
video.play();
```
to the end of the hydration function that the Svelte compiler emits for the component, I sometimes (though not always, which seems to imply that whatever is pausing the video is being called asynchronously) get the error [1]:
```
Uncaught (in promise) DOMException: The play() request was interrupted by a call to pause().
```
and the video remains paused in all cases. If, however, I add a simple timeout to that line:
```js
setTimeout(() => {
video.play();
}, 0);
```
the video plays, which seems to rule out the hydration function as the culprit.
This bug only shows up in Chrome (tested in version 70.0.3538.77). Safari and Firefox don't seem to be affected.
I've set up a Sapper project that demonstrates this [here](https://github.com/nsivertsen/svelte-video-autoplay-bug) and a Glitch project with a live demo [here](https://nsivertsen-svelte-video-autoplay-bug.glitch.me/). I've added the video to the "Home" and "About" pages. Whichever one you load first should show a paused video, and when you navigate to the other page it should play.
---
1. More info on the Google Developers site: [DOMException: The play() request was interrupted](https://developers.google.com/web/updates/2017/06/play-request-was-interrupted) | feature request,stale-bot,temp-stale | low | Critical |
376,027,210 | TypeScript | Infer-from-usage should find references of related tokens | Based on code in acorn:
```js
var TokenType = function TokenType(label, conf) {
this.label = label
this.binop = conf.binop || null
}
/**
* @param {string} name
* @param {number} prec
*/
function x(name, prec) {
return new TokenType(name, { binop: prec })
}
```
**Expected behavior:**
TokenType's inferred type should be `(label: string, conf: { binop: string })` based on usage in `x`.
**Actual behavior:**
TokenType's inferred type is `(label: any, conf: { binop: null })`, based only on usage inside `TokenType` itself.
I don't think infer-from-usage supports any JS assignment patterns; this is just the first one I ran into.
| Suggestion,Domain: Quick Fixes,Experience Enhancement,feature-request | low | Minor |
376,064,766 | go | cmd/go: align comments in go.mod files | Please answer these questions before submitting your issue. Thanks!
### What version of Go are you using (`go version`)?
Go 1.11
### Does this issue reproduce with the latest release?
yes
### What did you do?
go mod init
go mod tidy
### What did you expect to see?
Into go.mod, is it possible to have (like into comment into source code) align of `// indirect`
```
module myModule
require (
github.com/something v1.0.0
github.com/foo v1.0.0 // indirect
github.com/foo/bar v1.0.0 // indirect
)
```
### What did you see instead?
```
module myModule
require (
github.com/something v1.0.0
github.com/foo v1.0.0 // indirect
github.com/foo/bar v1.0.0 // indirect
)
```
| NeedsDecision,modules | low | Major |
376,096,611 | pytorch | batch_norm doesn't bump version counter of running stats | This can cause correctness problems if you mix `eval` and `training` modes because `eval` mode backward needs the running stats, and `training` mode forward updates the running stats, even if the `training` mode result is unused. E.g.,
```py
import torch
bn = torch.nn.BatchNorm2d(3).double()
x = torch.randn(2, 3, 1, 1).double().requires_grad_()
def f(x):
bn.running_mean.data.fill_(0)
bn.running_var.data.fill_(1)
bn.eval(); y0 = bn(x)
bn.train(); unused = bn(x) # running buffers are changed in-place here
return y0
torch.autograd.gradcheck(f, (x,)) # fails gradcheck
def g(x):
bn.running_mean.data.fill_(0)
bn.running_var.data.fill_(1)
bn.eval(); y0 = bn(x)
bn.running_mean = bn.running_mean.clone()
bn.running_var = bn.running_var.clone()
bn.train(); unused = bn(x)
return y0
torch.autograd.gradcheck(g, (x,)) # passes
```
cc @ezyang @gchanan @zou3519 @SsnL @albanD @gqchen @colesbury | high priority,module: autograd,module: nn,triaged,actionable,fixathon | medium | Major |
376,108,009 | pytorch | [caffe2] How to use Split operator? | Hi all,
how can I use the Split operator? I want to split a tensor and get the splitted blobs based on a indicated split number for further processing. Something like this:
```
...
relu = brew.relu(m, fc3, fc3)
split1, split2 = m.net.Split(relu, num_splits=2,axis=0)
conv2 = brew.conv(m, split1, 'conv2', .....................)
```
Is it possible? | caffe2 | low | Minor |
376,117,815 | TypeScript | Skip type checking project reference redirect(declaration file from project reference) to improve perf | <!-- π¨ STOP π¨ π¦π§π’π£ π¨ πΊπ»πΆπ· π¨
Half of all issues filed here are duplicates, answered in the FAQ, or not appropriate for the bug tracker. Please read the FAQ first, especially the "Common Feature Requests" section.
-->
## Search Terms
<!-- List of keywords you searched for before creating this issue. Write them down here so that others can find this suggestion more easily -->
## Suggestion
We could probably set internal option and skip type checking project reference redirects (included .d.ts files from project reference output)
This could speed up `tsc --b` mode by skipping type checking generated files, (eg. skip `compiler.d.ts` when building `tsc` project and so on...) Since in build mode referencing projects are not built if there are any errors in the referenced project this should report all errors.
## Use Cases
<!--
What do you want to use this for?
What shortcomings exist with current approaches?
-->
## Examples
<!-- Show how this would be used and what the behavior would be -->
## Checklist
My suggestion meets these guidelines:
* [ ] This wouldn't be a breaking change in existing TypeScript / JavaScript code
* [ ] This wouldn't change the runtime behavior of existing JavaScript code
* [ ] This could be implemented without emitting different JS based on the types of the expressions
* [ ] This isn't a runtime feature (e.g. new expression-level syntax)
| Suggestion,In Discussion,Scenario: Monorepos & Cross-Project References,Domain: Performance | low | Critical |
376,118,379 | rust | Specific implementations on wrapper types should show up on the page of the inner type | Currently, with the following code:
```rust
pub struct Rc<T>(T);
pub struct Foo;
impl Rc<Foo> {
pub fn bar() {}
}
```
the impl appears on the page for `Rc`, even though it's more relevant for `Foo`.
Provide a way to mark a type as a wrapper type, such that an impl where its first generic parameter is a single type (which may itself be generic, e.g. `Rc<Option<T>>`) appears on the page of that type.
It may be worth automatically doing this for `#[fundamental]` types | T-rustdoc,C-enhancement,A-trait-system,A-rustdoc-ui | low | Minor |
376,140,752 | react | Using both getDerivedStateFromError and getDerivedStateFromProps can be a foot gun | <!--
Note: if the issue is about documentation or the website, please file it at:
https://github.com/reactjs/reactjs.org/issues/new
-->
**Do you want to request a *feature* or report a *bug*?**
Feature
**What is the current behavior?**
If `componentDidCatch` and/or `getDerivedStateFromError` put the component in a state that avoids the cause of the error, and `getDerivedStateFromProps` reverts that state change, the error boundary will (obviously) fail to avert disaster.
https://codesandbox.io/s/pj0lwxk15j
It sounds very obvious when simplified like this, but when my team updated to React 16.5.x (and 16.6.0), suddenly this started happening for us. So something changed internally, but it's hard to pinpoint what. Unfortunately I haven't been able to create a small repro for that specific case that works in 16.4.2 but not 16.5.0 β so I'm making this issue about avoiding the cause in the first place.
**What is the expected behavior?**
Naively, my thinking is that because errors are more exceptional, let the state from error handlers take precedence. Probably there's a reason why this can't happen so a warning of some kind when this can happen would be nice. Two ways to do this comes to mind:
1. Cross-check that keys in state from `getDerivedStateFromProps` or `setState` in `componentDidCatch` don't collide with `getDerivedStateFromError` (sounds a bit far fetched?)
2. Be more explicit in documentation for error boundaries about how `getDerivedStateFromProps` will run last so that a developer can consider this case
**Which versions of React, and which browser / OS are affected by this issue? Did this work in previous versions of React?**
16.5.0 and up (16.4.2 behaves a bit differently βΒ see above), all platforms/browsers. | Type: Discussion | low | Critical |
376,153,252 | TypeScript | JSDoc doesn't dig through type aliases | **TypeScript Version:** 6fd6a04f2eabcbd468b80aac270b34bad24892e4
**Code**
```ts
/** @param containingNode Node to check for parse error */
type AddUnusedDiagnostic = (containingNode: number, type: number, diagnostic: number) => void;
```
**Expected behavior:** `containingNode` in the JSDoc comment binds to the parameter of the function type (e.g. rename, quick info, document highlights, and GTD work).
**Actual behavior:** `containingNode` doesn't bind to anything and the quick info just says it has type `any`. | Suggestion,Domain: JSDoc,Domain: Type Display,Experience Enhancement | low | Critical |
376,167,757 | TypeScript | Nested indexing of mapped type fails to resolve correctly | <!-- π¨ STOP π¨ π¦π§π’π£ π¨ πΊπ»πΆπ· π¨
Half of all issues filed here are duplicates, answered in the FAQ, or not appropriate for the bug tracker. Even if you think you've found a *bug*, please read the FAQ first, especially the Common "Bugs" That Aren't Bugs section!
Please help us by doing the following steps before logging an issue:
* Search: https://github.com/Microsoft/TypeScript/search?type=Issues
* Read the FAQ: https://github.com/Microsoft/TypeScript/wiki/FAQ
Please fill in the *entire* template below.
-->
<!-- Please try to reproduce the issue with `typescript@next`. It may have already been fixed. -->
**TypeScript Version:** 3.2.0-dev.20181031
<!-- Search terms you tried before logging this (so others can find this issue more easily) -->
**Search Terms:**
`nested indexing mapped type`
**Code**
```ts
interface Foo {
a: { x: boolean };
b: { y: boolean };
}
function extractBoolean<K extends 'a' | 'b'>(foo: Foo, key: K, innerKey: keyof Foo[K]): boolean {
return foo[key][innerKey];
}
```
**Expected behavior:**
Should compile.
**Actual behavior:**
```
Type 'Foo[K][keyof Foo[K]]' is not assignable to type 'boolean'.
Type '({ x: boolean; } | { y: boolean; })[keyof Foo[K]]' is not assignable to type 'boolean'.
```
[**Playground Link**](https://www.typescriptlang.org/play/#src=interface%20Foo%20%7B%0D%0A%20%20a%3A%20%7B%20x%3A%20boolean%20%7D%3B%0D%0A%20%20b%3A%20%7B%20y%3A%20boolean%20%7D%3B%0D%0A%7D%0D%0A%0D%0Afunction%20extractBoolean%3CK%20extends%20'a'%20%7C%20'b'%3E(foo%3A%20Foo%2C%20key%3A%20K%2C%20innerKey%3A%20keyof%20Foo%5BK%5D)%3A%20boolean%20%7B%0D%0A%20%20return%20foo%5Bkey%5D%5BinnerKey%5D%3B%0D%0A%7D%0D%0A)
**Related Issues:** <!-- Did you find other bugs that looked similar? -->
| Bug,Domain: Indexed Access Types | low | Critical |
376,179,505 | vscode | [css] Add @media and @supports to CSS breadcrumbs | It would be really useful if the navigation breadcrumbs for css included @media and @supports queries.
At the moment, if you are working inside a long media query, there's no easy way to tell aside from scrolling up and manually checking for one.
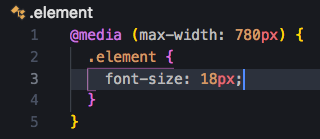
| feature-request,css-less-scss | low | Minor |
376,181,013 | TypeScript | Quick info for array in `a.length` shows `any[]` even when it should have a type | **TypeScript Version:** 3.2.0-dev.20181031
**Code**
```ts
/// <reference path='fourslash.ts'/>
// @strict: true
////function f(b: number) {
//// const /*0*/a = [];
//// if (b) /*1*/a.push(0);
//// /*2*/a.length;
//// /*3*/a.concat;
////}
verify.quickInfos({
0: "const a: any[]",
1: "const a: any[]",
2: "const a: any[]", // Should be number[]
3: "const a: number[]",
});
```
**Expected behavior:**
Quick info at `a` in `a.length` should show it as a `number[]`.
**Actual behavior:**
Shows `any[]`. | Bug,Domain: Quick Info | low | Minor |
376,190,692 | TypeScript | mixing generics, Pick, Exclude and intersection types fails to compile in 3.1.0 | <!-- π¨ STOP π¨ π¦π§π’π£ π¨ πΊπ»πΆπ· π¨
Half of all issues filed here are duplicates, answered in the FAQ, or not appropriate for the bug tracker. Even if you think you've found a *bug*, please read the FAQ first, especially the Common "Bugs" That Aren't Bugs section!
Please help us by doing the following steps before logging an issue:
* Search: https://github.com/Microsoft/TypeScript/search?type=Issues
* Read the FAQ: https://github.com/Microsoft/TypeScript/wiki/FAQ
Please fill in the *entire* template below.
-->
<!-- Please try to reproduce the issue with `typescript@next`. It may have already been fixed. -->
**TypeScript Version:** 3.2.0-dev.20181031
<!-- Search terms you tried before logging this (so others can find this issue more easily) -->
**Search Terms:** generic pick exclude keyof intersection spread
**Code**
```ts
type TWithFoo<T> = Pick<T, Exclude<keyof T, "foo">> & { foo: string };
const foobar: TWithFoo<{ foo: number; bar: string }> = {
foo: "foo",
bar: "bar",
};
function strictSpread<T, K extends keyof T>(
original: T,
override: Pick<T, K> | T
): T {
return Object.assign({}, original, override);
}
// Works as expected.
strictSpread(foobar, {
foo: "foo",
bar: "bar",
});
// Fails as expected.
strictSpread(foobar, {
baz: "foo",
});
function fn<T extends object>() {
const o: TWithFoo<T> = null as any;
// Fails unexpectedly.
strictSpread(o, {
foo: "foo",
});
}
```
**Expected behavior:**
The line annotated "fails unexpectedly" should compile successfully.
AFAICT this change happened between `3.1.0-dev.20180825` and `3.1.0-dev.20180828`.
**Actual behavior:**
The line annotated "fails unexpectedly" does not compile, though it used to in earlier versions.
The error reported is
```
Type '"foo"' is not assignable to type 'T["foo"] & string'.
Type '"foo"' is not assignable to type 'T["foo"]'.
```
But `TWithFoo` is specifically defined to ignore any existing `foo` key and replace it with `string`.
**Playground Link:** [link](https://www.typescriptlang.org/play/index.html#src=type%20TWithFoo%3CT%3E%20%3D%20Pick%3CT%2C%20Exclude%3Ckeyof%20T%2C%20%22foo%22%3E%3E%20%26%20%7B%20foo%3A%20string%20%7D%3B%0D%0A%0D%0Aconst%20foobar%3A%20TWithFoo%3C%7B%20foo%3A%20number%3B%20bar%3A%20string%20%7D%3E%20%3D%20%7B%0D%0A%20%20foo%3A%20%22foo%22%2C%0D%0A%20%20bar%3A%20%22bar%22%2C%0D%0A%7D%3B%0D%0A%0D%0Afunction%20strictSpread%3CT%2C%20K%20extends%20keyof%20T%3E(%0D%0A%20%20original%3A%20T%2C%0D%0A%20%20override%3A%20Pick%3CT%2C%20K%3E%20%7C%20T%0D%0A)%3A%20T%20%7B%0D%0A%20%20return%20Object.assign(%7B%7D%2C%20original%2C%20override)%3B%0D%0A%7D%0D%0A%0D%0A%2F%2F%20Works%20as%20expected.%0D%0AstrictSpread(foobar%2C%20%7B%0D%0A%20%20foo%3A%20%22foo%22%2C%0D%0A%20%20bar%3A%20%22bar%22%2C%0D%0A%7D)%3B%0D%0A%0D%0A%2F%2F%20Fails%20as%20expected.%0D%0AstrictSpread(foobar%2C%20%7B%0D%0A%20%20baz%3A%20%22foo%22%2C%0D%0A%7D)%3B%0D%0A%0D%0A%2F%2F%20Fails%20unexpectedly.%0D%0Afunction%20fn%3CT%20extends%20object%3E()%20%7B%0D%0A%20%20const%20o%3A%20TWithFoo%3CT%3E%20%3D%20null%20as%20any%3B%0D%0A%20%20strictSpread(o%2C%20%7B%0D%0A%20%20%20%20foo%3A%20%22foo%22%2C%0D%0A%20%20%7D)%3B%0D%0A%7D)
**Related Issues:** #27201, #27928
| Bug,Domain: Indexed Access Types | low | Critical |
376,196,280 | opencv | OpenGL code in samples/cpp/detect_mser.cpp | We should keep samples simple as much as possible.
What is OpenGL part demonstrate in this sample?
Can we port this code into OpenCV code without OpenGL usage?
Should we move original "OpenGL" code into "samples/opengl" sub-directory? | category: samples,RFC | low | Minor |
376,196,486 | go | x/tools/go/packages: failure parsing go.mod silently ignored | Followup from #28518.
We spent a while chasing our tails before realizing that `go list` was failing entirely. I think the error was swallowed because the call fell into the `usesExportData` case of `invokeGo`'s error handling. Can anything better be done?
@ianthehat @matloob | modules,Tools | low | Critical |
376,204,611 | flutter | Make BorderStyle extensible | It would be interesting to support new kinds of BorderStyle, based on some sort of interface that converts a Path to another Path for later processing.
e.g. to enable a package to implement dashed borders for the Border class and friends. | c: new feature,framework,customer: crowd,P3,team-framework,triaged-framework | medium | Major |
376,215,390 | rust | .count() too slow | I noticed something peculiar in #33038, notably
> So in short, the slowdown is caused by .count() using 64 bit integers. Which is strange, because the length of the vector is known be less than 2^32 - is there any way to optimize Vec or count() to use something smaller than usize?
In my understanding, `.count()` using `usize` directly contradicts
> So how do you know which type of integer to use? If youβre unsure, Rustβs defaults are generally good choices, and integer types default to i32: this type is generally the fastest, even on 64-bit systems. The primary situation in which youβd use isize or usize is when indexing some sort of collection.
from https://doc.rust-lang.org/book/second-edition/ch03-02-data-types.html
`.count()` is certainly never realistically being used for indexing, so according to the above paragraph it should default to `i32` on 64bit systems (This is referring to automatic typing of integer literals, but as an idea it remains valid). If this was already thoroughly discussed, I apologize. If not, I think it needs a second look. I think that in the majority of use cases, the users of Rust would much prefer `.count()` be three times faster, as opposed to being able to surpass 4 Billion. | A-LLVM,I-slow,T-compiler | low | Major |
376,223,890 | TypeScript | Compiler API to resolve path from ImportDeclaration | Hi there, since TS supports `paths` in `tsconfig.json`, is there an API in the compiler (`Program`, `CompilerHost` or something) to programmatically resolve real path of an import?
I wrote this transformer https://github.com/longlho/ts-transform-css-modules and it's tripping up on paths that are aliased in `tsconfig.json`, e.g:
```
import * as css from 'alias/foo.css'
```
tsconfig.json
```
{
"compilerOptions": {
"paths": {
"alias/*": "../../../some_location/*"
}
}
}
```
I can try to manually reconstruct TS resolver but rather not to.
Thanks! | Suggestion,In Discussion,API | low | Minor |
376,232,772 | react | Unexpected copies of the props object retained in memory with the new hooks API | <!--
Note: if the issue is about documentation or the website, please file it at:
https://github.com/reactjs/reactjs.org/issues/new
-->
**Do you want to request a *feature* or report a *bug*?**
bug
**What is the current behavior?**
```jsx
function useCustomHook1() {
useEffect(() => {
console.log("mounted");
return () => {
console.log("unmounted");
};
}, []);
}
function Test3(props) {
useCustomHook1();
useEffect(() => {
console.log(props.test);
});
return <div onClick={() => console.log(props.test)}>{props.items.length}</div>;
}
```
`props` object that were used when component was rendered for the first time is kept alive. Completely unexpected behavior even when developer understands [closure context sharing](https://mrale.ph/blog/2012/09/23/grokking-v8-closures-for-fun.html).
**If the current behavior is a bug, please provide the steps to reproduce and if possible a minimal demo of the problem. Your bug will get fixed much faster if we can run your code and it doesn't have dependencies other than React. Paste the link to your JSFiddle (https://jsfiddle.net/Luktwrdm/) or CodeSandbox (https://codesandbox.io/s/new) example below:**
https://codesandbox.io/s/lz61v39r7
- Select test **3**
- Click on the button "Create New Array" 5 times
- Take memory snapshot in the developer tools
- Inspect (array) objects
- There will be 4 arrays retained in memory `t0`, `t-2`, `t-1`, `t`
**What is the expected behavior?**
Should be 2 arrays retained in memory `t-1`, `t`. | Type: Needs Investigation,Component: Reconciler | low | Critical |
376,245,386 | neovim | :edit scrolls the viewport | <!-- Before reporting: search existing issues and check the FAQ. -->
- `nvim --version`: 0.3.1
- Vim (version: ) behaves differently? : cursor line does not move
- Operating system/version: ubuntu 14.04
- Terminal name/version: xterm
- `$TERM`: xterm-256color
### Steps to reproduce using `nvim -u NORC`
```
nvim -u NORC
:call setline(1, range(1, 1000))
:w /tmp/test.txt
:normal 500G
:normal zb
:edit # cursor moves here
```
### Actual behaviour
the screen scrolls and the cursor line moves to the middle of the screen
### Expected behaviour
the screen remains the same
### More information
according to Christian Brabandt 's comment to [the stackoverflow question](https://vi.stackexchange.com/questions/17780/neovim-cursor-line-moves-to-the-middle-of-the-window-after-edit), this patch [v8.1.0034](https://github.com/vim/vim/releases/tag/v8.1.0034) may has some effect | enhancement | low | Minor |
376,321,546 | TypeScript | Conflicting function return types checked for single bindings, but not overloads. | <!-- π¨ STOP π¨ π¦π§π’π£ π¨ πΊπ»πΆπ· π¨
Half of all issues filed here are duplicates, answered in the FAQ, or not appropriate for the bug tracker. Even if you think you've found a *bug*, please read the FAQ first, especially the Common "Bugs" That Aren't Bugs section!
Please help us by doing the following steps before logging an issue:
* Search: https://github.com/Microsoft/TypeScript/search?type=Issues
* Read the FAQ: https://github.com/Microsoft/TypeScript/wiki/FAQ
Please fill in the *entire* template below.
-->
<!-- Please try to reproduce the issue with `typescript@next`. It may have already been fixed. -->
**TypeScript Version:** 3.1.1-insiders.20180926 (what's running in the playground)
<!-- Search terms you tried before logging this (so others can find this issue more easily) -->
**Search Terms:** conflicting function return
**Code**
```ts
declare var foo: () => number
declare var foo: () => string
declare function bar(): number
declare function bar(): string
```
**Expected behavior:** `foo` and `bar` to both fail
**Actual behavior:** `bar` seems to still type-check, inconsistently with `foo`
**Playground Link:** https://www.typescriptlang.org/play/index.html#src=declare%20var%20foo%3A%20()%20%3D%3E%20number%0D%0Adeclare%20var%20foo%3A%20()%20%3D%3E%20string%0D%0A%0D%0Adeclare%20function%20bar()%3A%20number%0D%0Adeclare%20function%20bar()%3A%20string
**Related Issues:** N/A | Suggestion,In Discussion | low | Critical |
376,366,057 | flutter | All plugins are grabbed with Implementation rathar than Api which prevents accessing the plugin from existing apps | ## Steps to Reproduce
### TLDR;
- I created a flutter-plugin (With a static listener that your existing app can register itself to):
https://github.com/shehabic/dhplugin/blob/master/android/src/main/java/com/deliveryhero/pandora/dhplugin/DhpluginPlugin.java
- I create a module to consume this plugin
https://github.com/shehabic/dhmodule/blob/master/pubspec.yaml
- I created an app that uses this flutter plugin
https://github.com/shehabic/demo-app-android
- existing app registers itself to answer requests coming from the communication channel.
https://github.com/shehabic/demo-app-android/blob/master/app/src/main/java/com/shehabic/demoapp/HomeActivity.kt#L25
- The problem is here:
https://github.com/flutter/flutter/blob/master/packages/flutter_tools/gradle/flutter.gradle#L187
### More details
1. Create any flutter plugin e.g. a plugin that will always ask **your existing app** for data.
2. Assume that the heavy functionality of the collecting data is already in your Existing App.
3. Assume for that purpose you decided to add a listener in your native implementaion for your plugin on both Android and iOS, such that everytime a message arrives from your communication channel you ask the existing app via the `listener registered to answer requests`
4. Assume that you're importing this plugin through flutter `module` (The standard way), and your module has dependencies to many plugins.
5. Your app will only see code implemented in the flutter-module, but not any of the plugins.
6. The problem is at this point.
https://github.com/flutter/flutter/blob/master/packages/flutter_tools/gradle/flutter.gradle#L187
the following change solves the problem:
```
api pluginProject
```
<!-- If possible, paste the output of running `flutter doctor -v` here. -->
```
[β] Flutter (Channel master, v0.10.2-pre.131, on Mac OS X 10.14 18A391, locale en-DE)
β’ Flutter version 0.10.2-pre.131 at /Users/m.osman/development/flutter
β’ Framework revision 01c7081565 (8 hours ago), 2018-10-31 21:12:51 -0700
β’ Engine revision c79faed71c
β’ Dart version 2.1.0 (build 2.1.0-dev.8.0 bf26f760b1)
[β] Android toolchain - develop for Android devices (Android SDK 28.0.3)
β’ Android SDK at /Users/m.osman/Library/Android/sdk
β’ Android NDK location not configured (optional; useful for native profiling support)
β’ Platform android-28, build-tools 28.0.3
β’ Java binary at: /Applications/Android Studio.app/Contents/jre/jdk/Contents/Home/bin/java
β’ Java version OpenJDK Runtime Environment (build 1.8.0_152-release-1136-b06)
β’ All Android licenses accepted.
[β] iOS toolchain - develop for iOS devices (Xcode 10.1)
β’ Xcode at /Applications/Xcode.app/Contents/Developer
β’ Xcode 10.1, Build version 10B61
β’ ios-deploy 2.0.0
β’ CocoaPods version 1.5.3
[β] Android Studio (version 3.2)
β’ Android Studio at /Applications/Android Studio.app/Contents
β’ Flutter plugin version 29.1.1
β’ Dart plugin version 181.5656
β’ Java version OpenJDK Runtime Environment (build 1.8.0_152-release-1136-b06)
[β] VS Code (version 1.28.2)
β’ VS Code at /Applications/Visual Studio Code.app/Contents
β’ Flutter extension version 2.19.0
[!] Connected device
! No devices available
```
| tool,engine,t: gradle,a: existing-apps,P2,a: plugins,team-engine,triaged-engine | low | Minor |
376,392,631 | pytorch | Support gathering nested lists in DataParallel | ## π Feature
<!-- A clear and concise description of the feature proposal -->
The [current implementation](https://github.com/pytorch/pytorch/blob/ee628d64b9d2bc8ab26655982aa3a158859a99c0/torch/nn/parallel/scatter_gather.py#L46) of the `gather` function supports simple data structures like tensors and dictionaries but can not handle nested structures such as a list of lists.
How about supporting them as well?
## Motivation
<!-- Please outline the motivation for the proposal. Is your feature request related to a problem? e.g., I'm always frustrated when [...]. If this is related to another GitHub issue, please link here too -->
Complex networks don't always return simple outputs such as tensors. Consider the following situation where the outputs from the forward pass of a question answering network is a dictionary with keys `loss`, `answers` and `qids`. Notice that `answers` and `qids` are a list of lists.
```python
# Output from forward pass
{'loss': tensor(8.6422, device='cuda:0', grad_fn=<ThAddBackward>),
'answers': [['July 4', 'American Independence'], ['July 1', 'Canadian Independence']],
'qids': [['C28fbfa5abq#1', 'C28fbfa5abq#2'], ['C2c6ca8b089q#1', 'C2c6ca8b089q#2']}
```
While running this model over multiple GPUs using `nn.DataParallel`, the following is passed as input to the [gather function](https://github.com/pytorch/pytorch/blob/ee628d64b9d2bc8ab26655982aa3a158859a99c0/torch/nn/parallel/scatter_gather.py#L46)
```python
# Input to the `gather` function
[{'loss': tensor(8.6422, device='cuda:0', grad_fn=<ThAddBackward>),
'answers': [['July 4', 'American Independence'], ['July 1', 'Canadian Independence']],
'qids': [['C28fbfa5abq#1', 'C28fbfa5abq#2'], ['C2c6ca8b089q#1', 'C2c6ca8b089q#2']},
{'loss': tensor(8.7826, device='cuda:0', grad_fn=<ThAddBackward>),
'answers': [['September 16', 'Mexican Independence'], ['November 3', 'Panamanian Independence']],
'qids': [['C28fbfcdefab#1', 'C28fbfcdefab#2'], ['C2c623ef089q#1', 'C2c623ef089q#2']}]
```
Here's the expected output where the tensors and lists are concatenated together.
```python
# Expected behaviour
{'loss': tensor([8.6422, 8.7826], device='cuda:0', grad_fn=<ThAddBackward>),
'answers': [['July 4', 'American Independence'], ['July 1', 'Canadian Independence'], ['September 16', 'Mexican Independence'], ['November 3', 'Panamanian Independence']],
'qids': [['C28fbfa5abq#1', 'C28fbfa5abq#2'], ['C2c6ca8b089q#1', 'C2c6ca8b089q#2'], ['C28fbfcdefab#1', 'C28fbfcdefab#2'], ['C2c623ef089q#1', 'C2c623ef089q#2']]}
```
But this is what happens right now. The `map` clause cannot handle nested lists.
```python
# Current behaviour
{'loss': tensor([8.6422, 8.7826], device='cuda:0', grad_fn=<ThAddBackward>),
'answers': [['<map object at 0x2ad2445a7f60>', '<map object at 0x2ad2445a75f8>'], ['<map object at 0x2b6862d87358>', '<map object at 0x2b6862d87668>']],
'qids': [['<map object at 0x2b6862d87668>', '<map object at 0x2b6862d875c0>'], ['<map object at 0x2b6862d87438>', '<map object at 0x2b6862d87828>']]}
```
## Pitch
<!-- A clear and concise description of what you want to happen. -->
One solution is to explicitly handle merging of lists together. This basically boils down to adding an extra condition for handling lists in the `gather` function [here](https://github.com/pytorch/pytorch/blob/ee628d64b9d2bc8ab26655982aa3a158859a99c0/torch/nn/parallel/scatter_gather.py#L62).
```python
if isinstance(out, list):
return [item for output in outputs for item in output]
```
## Alternatives
<!-- A clear and concise description of any alternative solutions or features you've considered, if any. -->
One workaround is to avoid nested outputs from the forward pass in the first place. This is still a workaround and not a solution.
## Additional context
<!-- Add any other context or screenshots about the feature request here. -->
I'm not sure if this is the right way to handle the larger problem of handling complex data structures.
For example one can always come up with a more nested structure which might not work with the proposed solution either. Should pytorch allow the provision for custom `gather` and `scatter` functions as it has done with `collate_fn`? | triaged,module: data parallel | low | Major |
376,416,489 | rust | format!() ignores precision while formatting Debug | The `precision` field is ignored when formatting/printing `Debug` representation. Apparently, it *is* honored when formatting `Display` representation.
https://play.rust-lang.org/?version=nightly&mode=debug&edition=2015&gist=516b0c6222b4b351b69e45c15943821b | T-lang | low | Critical |
376,430,175 | go | cmd/go: -buildmode=pie does not build correct PIE binaries for ppc64le | Please answer these questions before submitting your issue. Thanks!
### What version of Go are you using (`go version`)?
latest
### Does this issue reproduce with the latest release?
yes
### What operating system and processor architecture are you using (`go env`)?
ppc64le
### What did you do?
Tried to run binaries built as PIE.
### What did you expect to see?
Correct execution
### What did you see instead?
Error messages like this:
./main.exe: error while loading shared libraries: R_PPC64_ADDR16_HA re10f2f8fa4 for symbol `' out of range
2018/09/05 09:53:14 Failed: exit status 127
on newer distros.
The problem is related to issues #27510 and #21954 and can still be reproduced on many distros by passing -pie as a linker option.
The solution to the above issues was to use -buildmode=pie but that doesn't correct all problems. When linking a program with -pie on ppc64le then all code should be built as PIC. When some code is not PIC there could be relocations created like R_PPC64_ADDR16_HA which will fail with the above error if the relocated addresses are too big. On some distros/kernels we get lucky and no failures occur if the addresses stay small enough but on newer distros the runtime relocation errors can occur.
When using -buildmode=pie the -shared option is set to force the compiles to be built as PIC but it still links in stdlib which was not built as PIC. Likewise any library linked in should be PIC. When all the code is has been built as PIC then this relocation would not be generated and the PIE should work.
One further question is whether all code should be PIC if the gcc being used for the link passes -pie by default and stop setting -no-pie. | NeedsInvestigation,arch-ppc64x | medium | Critical |
376,525,173 | TypeScript | Add new moduleResolution option: `yarn-pnp` | ## Search Terms
yarn pnp plug'n'play plug
## Suggestion
Yarn has released a new feature for using modules without having a `node_modules` directory present: plug'n'play. Some community tooling is available for customizing the TypeScript compilerHost so that it can use plug'n'play modules, but this does not work for users of `tsc`.
My suggestion is to add a new `"moduleResolution": "yarnpnp"` option.
## Use Cases / Examples
I want to be able to use yarn plug-n-play in my typescript projects. My projects are typically yarn monorepos where common libraries are basic node packages using `tsc` for compilation. This would allow the basic projects to continue using `tsc`, but with an alternative module resolution scheme.
Some community tooling has been created here for augmenting the CompileHost with a slightly updated moduleResolution strategy, but using it in basic projects would probably require a forked `tsc`.
https://github.com/arcanis/ts-pnp
## Checklist
My suggestion meets these guidelines:
* [x] This wouldn't be a breaking change in existing TypeScript / JavaScript code
* [x] This wouldn't change the runtime behavior of existing JavaScript code
* [x] This could be implemented without emitting different JS based on the types of the expressions
* [x] This isn't a runtime feature (e.g. new expression-level syntax)
| Suggestion,Awaiting More Feedback | high | Critical |
376,532,119 | godot | InputEvent's device id is always 0 |
**Godot version:** 3.0.5stable & 3.1alpha (13eaded)
**OS/device including version:** Linux 4.18.16
<!-- Specify GPU model and drivers if graphics-related. -->
**Issue description:** The eventβs device ID is always set to 0, was hoping to detect what keyboard or mouse was creating the InputEvent from in "func _input(event):..."
<!-- What happened, and what was expected. -->
**Steps to reproduce:** Add a script to a node and and have it access the InputEvents "device" property.
**Minimal reproduction project:**[InputEvents device id is always 0.zip](https://github.com/godotengine/godot/files/2539757/InputEvents.device.id.is.always.0.zip)
<!-- Recommended as it greatly speeds up debugging. Drag and drop a zip archive to upload it. -->
| bug,discussion,topic:porting,documentation,topic:input | low | Critical |
376,535,539 | rust | Build errors with edition=2018 after clean `cargo fix --edition` | With `nightly-2018-11-01` in Servo, after finally getting `cargo fix --edition` to complete without warnings. (Which was not easy: https://github.com/rust-lang-nursery/rustfix/issues/149, https://github.com/rust-lang-nursery/rustfix/issues/150), I added `edition = "2018"` to (almost) all `Cargo.toml` files.
At this point, the theory is that `cargo check` should Just Work, but it doesnβt. Iβve hit multiple issues, let me know if they should be filed separately.
## Dependencies not known to Cargo
```rust
error[E0658]: use of extern prelude names introduced with `extern crate` items is unstable (see issue #54658)
--> components/dom_struct/lib.rs:11:5
|
11 | use proc_macro::TokenStream;
| ^^^^^^^^^^
|
= help: add #![feature(extern_crate_item_prelude)] to the crate attributes to enable
```
The "extern prelude" is one way to fix this, but another is to is a crate-local path `crate::proc_macro::TokenStream` to the name that is introduced by `extern crate`.
This feature is also missing a tracking issue, the error message points to the implementation PR: https://github.com/rust-lang/rust/pull/54658#issuecomment-435156630
## Ambiguous imports
```rust
error: `time` import is ambiguous
--> components/compositing/compositor.rs:41:5
|
23 | use profile_traits::time::{self, ProfilerCategory, profile};
| ---- may refer to `self::time` in the future
...
41 | use time::{now, precise_time_ns, precise_time_s};
| ^^^^ can refer to external crate `::time`
|
= help: write `::time` or `self::time` explicitly instead
= note: in the future, `#![feature(uniform_paths)]` may become the default
error: aborting due to previous error
```
## Migrating generated code
`cargo fix` did fix similar cases in "normal" source files, but generated code needs to be fixed in the code generator.
These were the remaining ones after Iβd already taken care of the absolute paths.
```rust
error: expected expression, found reserved keyword `try`
--> /home/simon/servo2/target/debug/build/script-b336041ffdaa5d29/out/Bindings/AnalyserNodeBinding.rs:291:23
|
291 | match try!(crate::dom::bindings::codegen::Bindings::AudioNodeBinding::AudioNodeOptions::new(cx, val)) {
| ^^^ expected expression
```
```rust
error: expected pattern, found reserved keyword `async`
--> /home/simon/servo2/target/debug/build/script-b336041ffdaa5d29/out/Bindings/XMLHttpRequestBinding.rs:1766:57
|
1766 | fn Open_(&self, method: ByteString, url: USVString, async: bool, username: Option<USVString>, password: Option<USVString>) -> Fallible<()>;
| ^^^^^ expected pattern
error: aborting due to previous error
```
## New warnings
Not as much of an issue than build errors, but slightly unexpected: switching editions uncovered new `unused_mut` warnings that were not present before. Maybe this is because 2018 currently implies NLL / MIR-borrowck? | C-bug,F-rust_2018_preview,S-needs-repro,A-edition-2018 | low | Critical |
376,556,467 | TypeScript | Re-export class as part of a different namespace | This is similar to https://github.com/Microsoft/TypeScript/issues/4529, but that issue was closed before it got any real traction.
Setup:
````ts
// panel.ts
export class Panel {
constructor() {}
}
export namespace Panel {
export enum GridLineTypeFlags {
NONE = 0x00
}
export enum NormalizationType {
NONE,
SEPARATE_AXIS
}
}
export default Panel;
````
````ts
// panoramicpanel.ts
export class PanoramicPanel {
constructor() {}
public toString(): string {
return 'PanoramicPanel';
}
}
````
In the `serieschart.ts` file I'm trying to export the `PanoramicPanel` class in the same way as I'm exporting the `Panel` class and namespace, so both can be accessed in my application code as `new SeriesChart.PanoramicPanel()` and as a type.
The only way I found to make this work is the following, where using the `import export` syntax gives an error. Is there a reason why there would be an error?
(And because we have a lot of code which depends on the structure of this module, we unfortunately can not get rid of the namespaces.)
````ts
// serieschart.ts
import { Panel as PanelType } from './panel';
import { PanoramicPanel as PanoramicPanelType} from './panoramicpanel';
class SeriesChart {
}
namespace SeriesChart {
export import Panel = PanelType;
// export import PanoramicPanel = PanoramicPanelType; <- Error: PanoramicPanelType only refers to a type but is used as a namespace here
// export { PanoramicPanelType as PanoramicPanel} <- Error: Export declarations are not permitted in namespace
// this works, but is very counter intuitive
export type PanoramicPanel = PanoramicPanelType;
export const PanoramicPanel = PanoramicPanelType;
}
export default SeriesChart;
````
| Suggestion,In Discussion | low | Critical |
376,567,696 | pytorch | [jit] restrict promotion of single-element arguments to lists | We had a bug where a `len(Tensor t)` call in script was getting dispatched to `aten::len(Tensor[] t)`. We fixed it by adding single-element version, but there's no reason we should be promoting the tensor to a singleton list.
The argument promotion should only happen for integers, and only for certain ops like `aten::zeros`.
| oncall: jit | low | Critical |
376,568,696 | TypeScript | Broken IndexedDB event typings in 3.1.5 | <!-- π¨ STOP π¨ π¦π§π’π£ π¨ πΊπ»πΆπ· π¨
Half of all issues filed here are duplicates, answered in the FAQ, or not appropriate for the bug tracker. Even if you think you've found a *bug*, please read the FAQ first, especially the Common "Bugs" That Aren't Bugs section!
Please help us by doing the following steps before logging an issue:
* Search: https://github.com/Microsoft/TypeScript/search?type=Issues
* Read the FAQ: https://github.com/Microsoft/TypeScript/wiki/FAQ
Please fill in the *entire* template below.
-->
<!-- Please try to reproduce the issue with `typescript@next`. It may have already been fixed. -->
**TypeScript Version:** 3.1.5
<!-- Search terms you tried before logging this (so others can find this issue more easily) -->
**Search Terms:**
indexedDB
**Code**
```ts
let dbreq = indexedDB.open('test', 1);
dbreq.onsuccess = function (evt) {
let database = evt.target.result;
}
```
**Expected behavior:**
`evt.target.result` should be an IDBDatabase
**Actual behavior:**
TS2339: Property 'result' does not exist on type 'EventTarget'.
**Playground Link:** <!-- A link to a TypeScript Playground "Share" link which demonstrates this behavior -->
http://www.typescriptlang.org/play/index.html#src=let%20dbreq%20%3D%20indexedDB.open('test'%2C%201)%3B%0D%0A%0D%0Adbreq.onsuccess%20%3D%20function%20(evt)%20%7B%0D%0A%20%20%20%20let%20database%20%3D%20evt.target.result%3B%0D%0A%7D
**Related Issues:** <!-- Did you find other bugs that looked similar? -->
This is caused by the updates resulting from https://github.com/Microsoft/TypeScript/issues/25547
It works if you add a cast:
```ts
let dbreq = indexedDB.open('test', 1);
dbreq.onsuccess = function (evt) {
let database = (evt.target as IDBOpenDBRequest).result;
}
``` | Bug,Domain: lib.d.ts | medium | Critical |
376,606,009 | create-react-app | Extended configs appear to not be fixed | https://github.com/facebook/create-react-app/issues/5645#issuecomment-435201019 | issue: typescript | low | Minor |
376,621,925 | TypeScript | Strict type-checking on a per-file basis "@ts-strict" | ## Search Terms
strict per file
## Suggestion
Currently in the process of converting a moderate-sized javascript project to typescript. Would love to be able to enable strict type-checks on a per-file basis.
## Use Cases
Enabling strict mode for the entire project is a daunting task that presents a barrier to adoption (and using typescript without strict mode turned on is like locking the doors on a convertible....it's probably better than nothing, but it's not gonna keep you safe).
## Examples
```
//@ts-strict
```
## Checklist
My suggestion meets these guidelines:
* [X] This wouldn't be a breaking change in existing TypeScript / JavaScript code
* [X] This wouldn't change the runtime behavior of existing JavaScript code
* [X] This could be implemented without emitting different JS based on the types of the expressions
* [X] This isn't a runtime feature (e.g. new expression-level syntax)
| Suggestion,In Discussion | high | Critical |
376,625,469 | pytorch | How to do inference with float16 (or half) in caffe2? | I cannot find any resource about running inference with float16. There is resnet.py for training with float16, but no information is provided for inference with float16.
Any help?
Thank you | caffe2 | low | Minor |
376,629,966 | go | runtime: unknown pc during parallel benchmarks | Please answer these questions before submitting your issue. Thanks!
### What version of Go are you using (`go version`)?
go1.11.1 linux/arm64
### Does this issue reproduce with the latest release?
yes
### What operating system and processor architecture are you using (`go env`)?
I'm using archlinux installed into a chroot on a chromebook, which is arm64.
### What did you do?
This error is quite easy for me to reproduce, but difficult to pin down a small test-case for it.
The following will cause the error, but requires a redis server to be running in order to do so:
```
curl https://gist.githubusercontent.com/mediocregopher/5b365a3dfedb58dfff5e7dc973dee480/raw/761d04ae1ebd44f2d806aa33bad16dde38d8e401/panic_test.go > panic_test.go
go get -u github.com/mediocregopher/radix.v3
go test -v -run xxx -bench Panic -benchmem
```
### What did you expect to see?
Both the `panicking` and `not-panicking` benchmarks to complete successfully.
### What did you see instead?
If I run that with `CGO_ENABLED=1` the runtime panics with an `unknown pc` error:
[with_cgo.txt](https://github.com/golang/go/files/2540666/with_cgo.txt)
If I run it with `CGO_ENABLED=0` it panics with an `unexpected fault address`: [without_cgo.txt](https://github.com/golang/go/files/2540661/without_cgo.txt)
Either way it seems the panicking is happening within the benchmark package, not my own, though I'm not able to find a reproducible test case without using my own code.
| NeedsInvestigation,compiler/runtime | low | Critical |
376,665,752 | pytorch | Caffe2 missing headers in docker/conda | ## π Bug
The conda environment that is part of the docker image is missing a bunch of headers
## To Reproduce
Steps to reproduce the behavior:
1. Create a docker image using the steps on the readme.
1. Try to include #include <caffe2/core/predictor.h>
```
/workspace/src/main.cpp:9:35: fatal error: caffe2/core/predictor.h: No such file or directory
compilation terminated.
```
## Expected behavior
I expect the header files to be present.
## Environment
```
PyTorch version: 1.0.0a0+4fe8ca7
Is debug build: No
CUDA used to build PyTorch: 9.0.176
OS: Ubuntu 16.04.5 LTS
GCC version: (Ubuntu 5.4.0-6ubuntu1~16.04.10) 5.4.0 20160609
CMake version: version 3.5.1
Python version: 3.6
Is CUDA available: Yes
CUDA runtime version: 9.0.176
GPU models and configuration: GPU 0: GeForce GTX 960M
Nvidia driver version: 410.66
cuDNN version: Probably one of the following:
/usr/lib/x86_64-linux-gnu/libcudnn.so.7.3.1
/usr/lib/x86_64-linux-gnu/libcudnn_static_v7.a
Versions of relevant libraries:
[pip] numpy (1.15.3)
[pip] torch (1.0.0a0+4fe8ca7)
[pip] torchvision (0.2.1)
[conda] magma-cuda90 2.3.0 1 pytorch
[conda] torch 1.0.0a0+4fe8ca7 <pip>
[conda] torchvision 0.2.1 <pip>
```
- Any other relevant information: Running in a docker container
## Additional context
Other headers are similarly missing. For example caffe2/threadpool/Threadpool.h
| caffe2 | low | Critical |
376,669,078 | go | x/build/kubernetes/gke: investigate if viable to get 4 tests to run on builders again | <!-- Please answer these questions before submitting your issue. Thanks! -->
### What version of Go are you using (`go version`)?
<pre>
$ go version
go version go1.11.1 linux/amd64
</pre>
### Does this issue reproduce with the latest release?
Yes.
### What operating system and processor architecture are you using (`go env`)?
<details><summary><code>go env</code> Output</summary><br><pre>
$ go env
GOARCH="amd64"
GOBIN=""
GOCACHE="/home/dmitshur/.cache/go-build"
GOEXE=""
GOFLAGS=""
GOHOSTARCH="amd64"
GOHOSTOS="linux"
GOOS="linux"
GOPATH="/home/dmitshur/go"
GOPROXY=""
GORACE=""
GOROOT="/usr/local/go"
GOTMPDIR=""
GOTOOLDIR="/usr/local/go/pkg/tool/linux_amd64"
GCCGO="gccgo"
CC="gcc"
CXX="g++"
CGO_ENABLED="1"
GOMOD=""
CGO_CFLAGS="-g -O2"
CGO_CPPFLAGS=""
CGO_CXXFLAGS="-g -O2"
CGO_FFLAGS="-g -O2"
CGO_LDFLAGS="-g -O2"
PKG_CONFIG="pkg-config"
GOGCCFLAGS="-fPIC -m64 -pthread -fmessage-length=0 -fdebug-prefix-map=/tmp/go-build290116553=/tmp/go-build -gno-record-gcc-switches"
</pre></details>
### What did you do?
<!--
If possible, provide a recipe for reproducing the error.
A complete runnable program is good.
A link on play.golang.org is best.
-->
I ran `go test golang.org/x/build/...` on GCE.
Note this doesn't happen in other environments, because those tests get skipped on non-GCE. E.g., here's verbose test output on my Mac:
```
$ go test -v golang.org/x/build/kubernetes/gke
=== RUN TestNewClient
--- SKIP: TestNewClient (0.05s)
gke_test.go:119: not on GCE; skipping
=== RUN TestDialPod
--- SKIP: TestDialPod (0.00s)
gke_test.go:119: not on GCE; skipping
=== RUN TestDialService
--- SKIP: TestDialService (0.00s)
gke_test.go:119: not on GCE; skipping
=== RUN TestGetNodes
--- SKIP: TestGetNodes (0.00s)
gke_test.go:119: not on GCE; skipping
PASS
ok golang.org/x/build/kubernetes/gke 0.103s
```
### What did you expect to see?
All tests to pass.
### What did you see instead?
```
--- FAIL: TestNewClient (0.67s)
gke_test.go:154: x509 client key pair could not be generated: tls: failed to find any PEM data in certificate input
--- FAIL: TestDialPod (0.60s)
gke_test.go:154: x509 client key pair could not be generated: tls: failed to find any PEM data in certificate input
--- FAIL: TestDialService (0.60s)
gke_test.go:154: x509 client key pair could not be generated: tls: failed to find any PEM data in certificate input
--- FAIL: TestGetNodes (0.59s)
gke_test.go:154: x509 client key pair could not be generated: tls: failed to find any PEM data in certificate input
FAIL
FAIL golang.org/x/build/kubernetes/gke 2.519s
``` | Builders,NeedsInvestigation | low | Critical |
376,670,456 | TypeScript | AudioWorkletProcessor type definition is missing | <!-- π¨ STOP π¨ π¦π§π’π£ π¨ πΊπ»πΆπ· π¨
Half of all issues filed here are duplicates, answered in the FAQ, or not appropriate for the bug tracker. Even if you think you've found a *bug*, please read the FAQ first, especially the Common "Bugs" That Aren't Bugs section!
Please help us by doing the following steps before logging an issue:
* Search: https://github.com/Microsoft/TypeScript/search?type=Issues
* Read the FAQ: https://github.com/Microsoft/TypeScript/wiki/FAQ
Please fill in the *entire* template below.
-->
<!-- Please try to reproduce the issue with `typescript@next`. It may have already been fixed. -->
**TypeScript Version:** 3.2.0-dev.201xxxxx
<!-- Search terms you tried before logging this (so others can find this issue more easily) -->
**Search Terms:** AudioWorkletProcessor
You guys have type definitions for AudioWorkletNode and such but missed the definition for AudioWorkletProcessor as per: https://webaudio.github.io/web-audio-api/#audioworkletprocessor
This is what I have for now:
```typescript
interface AudioWorkletProcessor {
readonly port: MessagePort;
process(inputs: Float32Array[][], outputs: Float32Array[][], parameters: Map<string, Float32Array>): void;
}
declare var AudioWorkletProcessor: {
prototype: AudioWorkletProcessor;
new(options?: AudioWorkletNodeOptions): AudioWorkletProcessor;
}
```
Cheers
| Bug,Help Wanted,Domain: lib.d.ts | medium | Critical |
376,697,584 | go | os: add test for Windows Dedup volumes | In recent CL 143578, I was going to remove some code that I thought did nothing. My change would have made all files on Windows Dedup volumes ( https://docs.microsoft.com/en-us/windows-server/storage/data-deduplication/overview ) look like symlinks.
Luckily @kolyshkin pointed my mistake ( https://github.com/golang/go/issues/22579#issuecomment-428381201 ). I built Windows instance on Gogle Cloud with extra disk attached as D: drive and with Dedup option on, to test my CL.
I wonder, if we can have one of our Windows builders have small extra disk with Dedup on. Then we could write some appropriate tests that run on that builder.
/cc @bradfitz @johnsonj
Alex | Testing,help wanted,OS-Windows,Builders,FeatureRequest,new-builder | low | Minor |
376,717,125 | opencv | Persistence refactoring: CUDA code is broken | [Build](http://pullrequest.opencv.org/buildbot/builders/master-contrib_cuda-lin64/builds/36).
relates #13011 | bug,priority: low,category: build/install,category: gpu/cuda (contrib) | low | Critical |
376,735,620 | TypeScript | Parser API: Expose a Method to Determine Whether a Numeric Literal is of Certain Flag | <!-- π¨ STOP π¨ π¦π§π’π£ π¨ πΊπ»πΆπ· π¨
Half of all issues filed here are duplicates, answered in the FAQ, or not appropriate for the bug tracker. Please read the FAQ first, especially the "Common Feature Requests" section.
-->
## Search Terms
Parser API numericLiteralFlags numeric literal flag
<!-- List of keywords you searched for before creating this issue. Write them down here so that others can find this suggestion more easily -->
## Suggestion
Currently the parser API apparently does not expose `numericLiteralFlags` for determining whether the original source is written using features such as binary or octal literal.
I have to do this to determine whether the original source was written using ECMAScript 2015 numeric literal:
```ts
if (TypeScript.isNumericLiteral(node)) {
let bitflag = node['numericLiteralFlags'];
if (bitflag) {
if (bitflag & (1 << 8)) {
// internal flag: Octal
// https://github.com/Microsoft/TypeScript/blob/a4a1bed88bdcb160eff032790f05629f9fa955b4/src/compiler/types.ts#L1659
return true;
}
if (bitflag & (1 << 7)) {
// internal flag: Binary
// https://github.com/Microsoft/TypeScript/blob/a4a1bed88bdcb160eff032790f05629f9fa955b4/src/compiler/types.ts#L1658
return true;
}
}
}
```
AFAIK this is the only way to check it since `node.text` evaluates to the string representation of the base 10 number...
## Use Cases
I need this 'internal' API to write [a parser for helping determine whether a JS file is compatible with syntax of certain ECMAScript version](https://github.com/ryanelian/instapack/blob/terser/src/SyntaxLevelChecker.test.ts). For example: Can this script run in ES5 environments?
`node_modules` may contain packages not distributed in ES5, which can cause minification error when being minified with minifier not capable of handling ES5, or worse, ninja runtime error. (I want to parse packages / libraries with TypeScript API, then transpile them only when necessary for fast build. Rather than transpiling everything)
<!--
What do you want to use this for?
What shortcomings exist with current approaches?
-->
## Examples
<!-- Show how this would be used and what the behavior would be -->
Simple proposed API:
```
isES2015OctalNumericLiteral(node)
isES2015BinaryNumericLiteral(node)
```
## Checklist
My suggestion meets these guidelines:
* [x] This wouldn't be a breaking change in existing TypeScript / JavaScript code
* [x] This wouldn't change the runtime behavior of existing JavaScript code
* [x] This could be implemented without emitting different JS based on the types of the expressions
* [x] This isn't a runtime feature (e.g. new expression-level syntax)
| Suggestion,In Discussion,API | low | Critical |
376,745,726 | opencv | python: gen2.py generates constructors for abstract interfaces | - OpenCV => 3.4 / master (probably all 3.x versions)
- Operating System / Platform => Windows 64 Bit
- Compiler => python3.5, opencv3.4.3 (from pip here)
##### Detailed description
we *should* use `XXX_create()` functions, but folks will try to use `XXX()` instead ,
leading to cryptic errors:
```
>>> img = np.array((2,2),np.uint8) # a dummy img, just for demo purpose
>>> o = cv2.ORB() # this should not be possible, but it is !
>>> o # it is a valid instance
<ORB 0000000603D44F90>
>>> o.detect(img)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: Incorrect type of self (must be 'Feature2D' or its derivative)
>>> type(o) # python thinks, everything is ok here ;(
<class 'cv2.ORB'>
>>> isinstance(o, cv2.Feature2D) # same delusion
True
>>>
>>> s = cv2.xfeatures2d.SIFT() # proper error.
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
AttributeError: module 'cv2.xfeatures2d' has no attribute 'SIFT'
>>> s = cv2.xfeatures2d_SIFT() # but uhhh !
>>> s.detect(img)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: Incorrect type of self (must be 'Feature2D' or its derivative)
>>>
>>> c = cv2.CLAHE() # here, too !
>>> c.apply(img)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: Incorrect type of self (must be 'CLAHE' or its derivative)
``` | bug,category: python bindings,effort: β | low | Critical |
376,771,004 | godot | Godot cut bottom of last item until show scroll | **Godot version:**
3.1 0e27af2
**OS/device including version:**
Windows 10
**Issue description:**
When window has a little smaller height than all elements need, Godot cut a some of pixels at the last item instead show scroll.
**Steps to reproduce:**
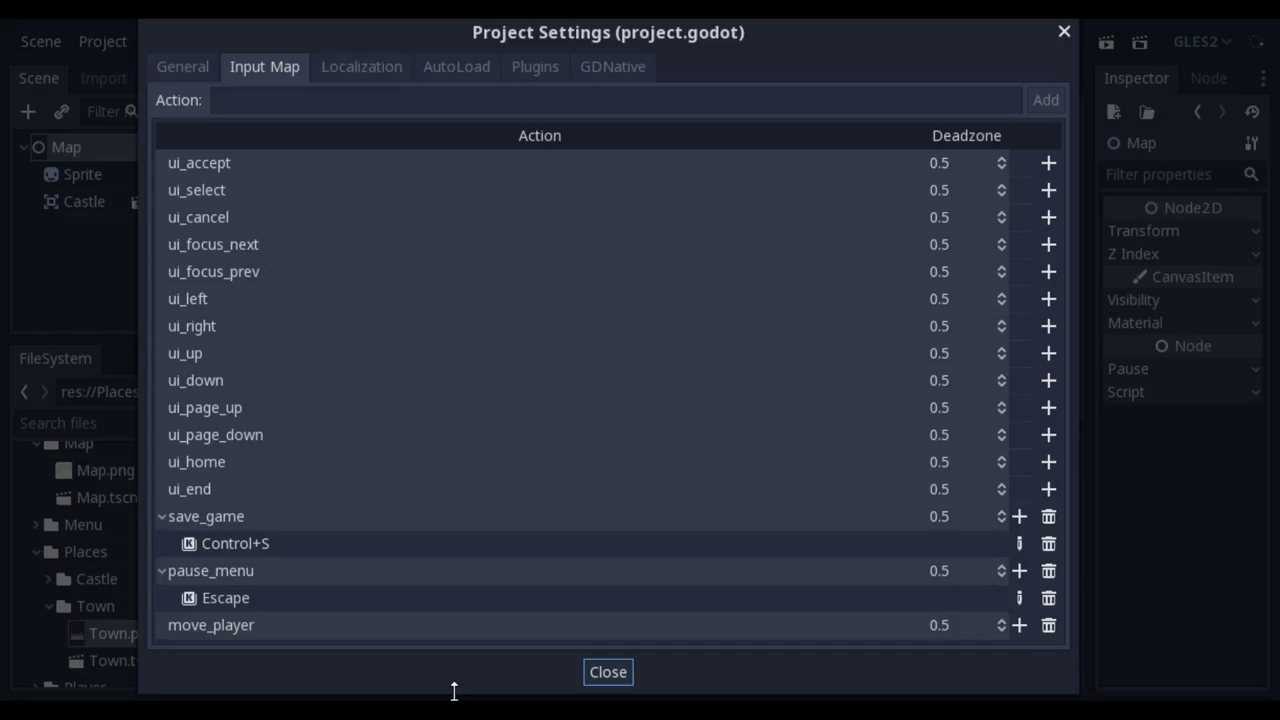
| bug,topic:editor,confirmed | low | Minor |
376,796,523 | pytorch | [feature request] Singular values and spectral norm for convolutional layers | Google Brain open-sourced a simple impl based on FFT: https://github.com/brain-research/conv-sv/blob/master/conv2d_singular_values.py ([paper](https://arxiv.org/abs/1805.10408))
It could be a nice addition to SpectralNorm or for debugging in general
cc @mruberry @peterbell10 @vincentqb @vishwakftw @jianyuh @nikitaved @pearu @SsnL | module: convolution,triaged,module: linear algebra,module: fft,function request | low | Critical |
376,804,446 | kubernetes | Disable cpu quota(use only cpuset) for pod Guaranteed | <!-- Please only use this template for submitting enhancement requests -->
**What would you like to be added**:
Disable cpu quota when we use cpuset for guaranteed pods.
**Why is this needed**:
For now, kubelet default adds cpu quota and share for all cases when `cpuCFSQuota` is open, even if *cpuset* will be set in guaranteed pod case.
However, this kind of use(cpuset with quota) may sometimes bring us cpu throttle time which we don't expect.
My question is why not make a `if` statement when we add cpuQuota config, if it's *guaranteed* case, just set `cpuQuota=-1`?
```
if m.cpuCFSQuota {
// if cpuLimit.Amount is nil, then the appropriate default value is returned
// to allow full usage of cpu resource.
cpuQuota, cpuPeriod := milliCPUToQuota(cpuLimit.MilliValue())
lc.Resources.CpuQuota = cpuQuota
lc.Resources.CpuPeriod = cpuPeriod
}
```
<!-- DO NOT EDIT BELOW THIS LINE -->
/kind feature | kind/bug,priority/backlog,sig/node,triage/accepted | high | Critical |
376,824,906 | pytorch | OpenCL support for smartphones | ## π Feature
OpenCL can be used to run AI at Mali GPUs present in top Exynos ARM chips in smart phones. I would be nice to run simple NN like [MTCNN](https://kpzhang93.github.io/MTCNN_face_detection_alignment/) locally without sending images to cloud.
## Motivation
OpenCL is supported by many top smart phones.
## Pitch
It would be nice to used embedded GPUs for AI.
## Alternatives
There is already some implementation of OpenCL tested at Mali GPUs here:
https://github.com/pmarcinkiew/pytorch/tree/opencl_release_1.0
You just need flag for OpenCL:
```
cmake . -DUSE_OPENCL=ON
```
It is necessary to have OpenCL libraries in your system for it to work correctly.
## Additional context
There is some OpenCL code already here:
https://github.com/pytorch/pytorch/tree/master/caffe2/contrib/opencl
but it doesn't compile by default and there are not operators (e.g. convolution)
| triaged | low | Minor |
376,825,812 | vscode | Setup has detected that setup is currently running |
Issue Type: <b>Bug</b>
Unexpected since I did not run any setup myself. Shortly before that the (1) badge showed up on the gear icon.
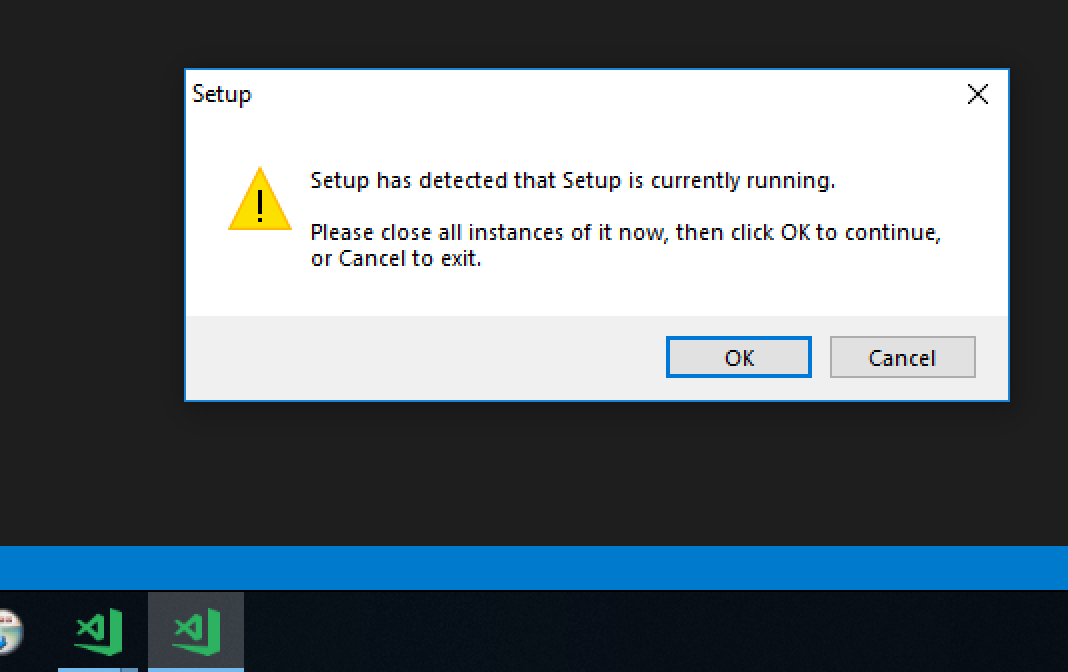
VS Code version: Code - Insiders 1.29.0-insider (220bff273eed39013f24b6d26fc17f2a742ce10f, 2018-11-02T06:18:20.695Z)
OS version: Windows_NT x64 10.0.17134
<details>
<summary>System Info</summary>
|Item|Value|
|---|---|
|CPUs|Intel(R) Core(TM) i7-4770HQ CPU @ 2.20GHz (4 x 2195)|
|GPU Status|2d_canvas: enabled<br>checker_imaging: disabled_off<br>flash_3d: enabled<br>flash_stage3d: enabled<br>flash_stage3d_baseline: enabled<br>gpu_compositing: enabled<br>multiple_raster_threads: enabled_on<br>native_gpu_memory_buffers: disabled_software<br>rasterization: unavailable_software<br>video_decode: enabled<br>video_encode: enabled<br>webgl: enabled<br>webgl2: enabled|
|Memory (System)|5.98GB (2.91GB free)|
|Process Argv|--inspect-extensions=51039|
|Screen Reader|no|
|VM|100%|
</details><details><summary>Extensions (15)</summary>
Extension|Author (truncated)|Version
---|---|---
remotehub|eam|0.2.0
EditorConfig|Edi|0.12.5
vscode-azureappservice|ms-|0.9.1
vscode-azurefunctions|ms-|0.12.0
vscode-azurestorage|ms-|0.4.2
vscode-cosmosdb|ms-|0.8.0
azure-account|ms-|0.4.3
azurecli|ms-|0.4.2
csharp|ms-|1.17.0
github-issues-prs|ms-|0.9.1
vscode-node-azure-pack|ms-|0.0.4
azurerm-vscode-tools|msa|0.4.2
debugger-for-chrome|msj|4.10.2
vscode-docker|Pet|0.3.1
vscode-open-in-github|sys|1.7.0
</details>
<!-- generated by issue reporter --> | bug,install-update,windows | high | Critical |
376,834,056 | go | cmd/link: suspicious use of (*cmd/internal/bio/buf.Reader).Seek | While a running a recent test of vet, it flagged the (*buf.Reader).Seek method as not having a proper return type (int64, error). Instead it handles errors by calling log.Fatal, yet most callers of this function seem to assume it returns a negative number to indicate failure.
Someone who knows this code should probably audit it.
| help wanted,NeedsInvestigation,compiler/runtime | low | Critical |
376,844,653 | TypeScript | Typeguards with destructuring parameters | ## Search Terms
Typeguard
type narrowing
destructuring
## Suggestion
It'd be nice to be able to have typeguard functions with destructuring arguments.
## Use Cases
When manipulating tuples or other anonymous structured types, whose typeguard relies only on a small part of the object, it would make the code much easier to read.
Currently, typeguards cannot be used with destructuring arguments, which makes it a weird special case.
## Examples
```ts
const val: Array<[string, boolean]> = ([] as Array<[string | undefined, boolean]>)
.filter(([foo, bar]: [string | undefined, boolean]): [foo, bar] is [string, boolean] => foo !== undefined);
const val2: Array<[string, boolean]> = ([] as Array<[string | undefined, boolean]>)
.filter((val: [string | undefined, boolean]): val is [string, boolean] => val[0] !== undefined);
```
https://www.typescriptlang.org/play/index.html#src=const%20val%3A%20Array%3C%5Bstring%2C%20boolean%5D%3E%20%3D%20(%5B%5D%20as%20Array%3C%5Bstring%20%7C%20undefined%2C%20boolean%5D%3E)%0D%0A%20%20%20%20.filter((%5Bfoo%2C%20bar%5D%3A%20%5Bstring%20%7C%20undefined%2C%20boolean%5D)%3A%20%5Bfoo%2C%20bar%5D%20is%20%5Bstring%2C%20boolean%5D%20%3D%3E%20foo%20!%3D%3D%20undefined)%3B%0D%0A%0D%0Aconst%20val2%3A%20Array%3C%5Bstring%2C%20boolean%5D%3E%20%3D%20(%5B%5D%20as%20Array%3C%5Bstring%20%7C%20undefined%2C%20boolean%5D%3E)%0D%0A%20%20%20%20.filter((val%3A%20%5Bstring%20%7C%20undefined%2C%20boolean%5D)%3A%20val%20is%20%5Bstring%2C%20boolean%5D%20%3D%3E%20val%5B0%5D%20!%3D%3D%20undefined)%3B%0D%0A%0D%0Aconst%20val3%3A%20string%5B%5D%20%3D%20(%5B%5D%20as%20Array%3Cstring%20%7C%20undefined%3E)%0D%0A%20%20%20%20.filter((foo%3F%3A%20string)%20%3D%3E%20foo%20!%3D%3D%20undefined)%3B%0D%0A
## Checklist
My suggestion meets these guidelines:
* [x] This wouldn't be a breaking change in existing TypeScript / JavaScript code
* [x] This wouldn't change the runtime behavior of existing JavaScript code
* [x] This could be implemented without emitting different JS based on the types of the expressions
* [x] This isn't a runtime feature (e.g. new expression-level syntax)
| Suggestion,Awaiting More Feedback | low | Minor |
376,856,350 | scrcpy | Multi-tasking windows | It would be great to be able to display each app in a separate window, similarly to Samsung DeX/Sentio/etc. | feature request | low | Minor |
376,881,605 | vscode | Not searching some files that aren't in an open folder | Issue Type: <b>Bug</b>
I've been having this problem for a few weeks - it used to work correctly. I have tested without extensions and it still occurs.
I open a set of log files (not a folder) and want to search all of them for errors. There are files with *.err file extensions which are repeatedly not getting searched in unless I click to focus them.
Precondition:
* Create some log files, including one named 'Daily_MR_EX_Load_20181102.err' with some text contents
Steps:
* Open VSCode
* Select several log files (including the one above) and drag them into the VSCode program window
* Without focusing on the .err log file, search for text that it should contain. It should not appear in the results.
* Then, focus on the .err file
* Search again, and the results should change.
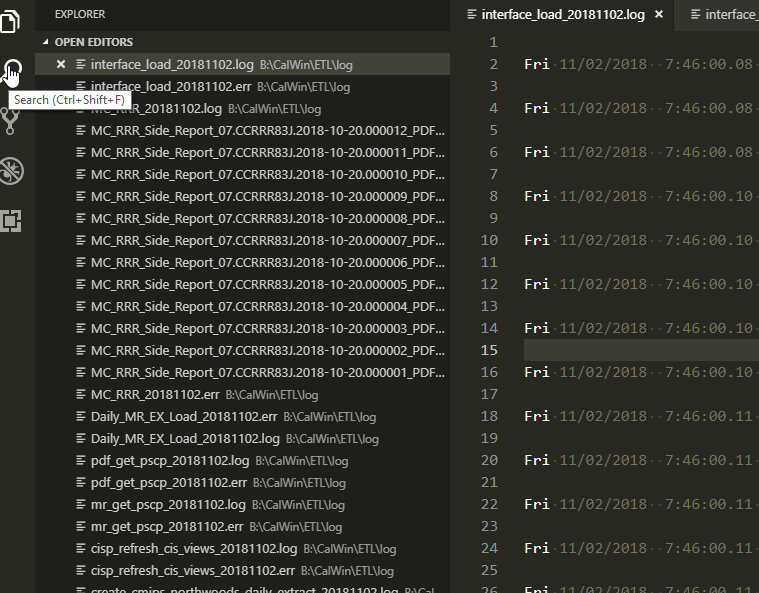
VS Code version: Code - Insiders 1.29.0-insider (9b2a7f4a83ca6a2d495463276a21157f398b41e7, 2018-11-02T13:42:21.142Z)
OS version: Windows_NT x64 10.0.15063
<details>
<summary>System Info</summary>
|Item|Value|
|---|---|
|CPUs|AMD A8-7650K Radeon R7, 10 Compute Cores 4C+6G (4 x 3292)|
|GPU Status|2d_canvas: enabled<br>checker_imaging: disabled_off<br>flash_3d: enabled<br>flash_stage3d: enabled<br>flash_stage3d_baseline: enabled<br>gpu_compositing: enabled<br>multiple_raster_threads: enabled_on<br>native_gpu_memory_buffers: disabled_software<br>rasterization: enabled<br>video_decode: enabled<br>video_encode: enabled<br>webgl: enabled<br>webgl2: enabled|
|Memory (System)|14.94GB (4.25GB free)|
|Process Argv||
|Screen Reader|no|
|VM|0%|
</details><details><summary>Extensions (6)</summary>
Extension|Author (truncated)|Version
---|---|---
gitlens|eam|8.5.6
gc-excelviewer|Gra|2.1.26
git-tree-compare|let|1.4.0
rainbow-csv|mec|0.7.1
mssql|ms-|1.4.0
vscode-spotify|shy|3.0.4
</details>
<!-- generated by issue reporter --> | bug,info-needed,search,confirmed | low | Critical |
376,910,018 | vscode | Keyboard Shortcuts Ide Tab and Browser Pdf Shift+Alt+... entries |
Issue Type: <b>Bug</b>
The Keyboard Shortcuts ide tab [ Ctrl+K Ctrl+S ] and browser pdf [ Ctrl+K Ctrl+R ] references have a bunch of Shift+Alt+... entries. For whatever mental reason I, and i'm guessing others, find these really confusing given historically we are used to seeing keyboard shortcuts involving use of Shift key listed as Ctrl+Shift+... and Alt+Shift+.... My recommendation here is that the 1/2 dozen Shift+Alt+... entries i'm seeing in these keyboard shortcut references be updated to use the more expected Alt+Shift+... format unless there was an intentional reason for using non-typical ordering.
VS Code version: Code 1.28.2 (7f3ce96ff4729c91352ae6def877e59c561f4850, 2018-10-17T00:23:51.859Z)
OS version: Windows_NT x64 10.0.17763
<details>
<summary>System Info</summary>
|Item|Value|
|---|---|
|CPUs|Intel(R) Core(TM) i7-8650U CPU @ 1.90GHz (8 x 2112)|
|GPU Status|2d_canvas: enabled<br>checker_imaging: disabled_off<br>flash_3d: enabled<br>flash_stage3d: enabled<br>flash_stage3d_baseline: enabled<br>gpu_compositing: enabled<br>multiple_raster_threads: enabled_on<br>native_gpu_memory_buffers: disabled_software<br>rasterization: enabled<br>video_decode: enabled<br>video_encode: enabled<br>webgl: enabled<br>webgl2: enabled|
|Memory (System)|15.85GB (6.41GB free)|
|Process Argv||
|Screen Reader|no|
|VM|0%|
</details><details><summary>Extensions (6)</summary>
Extension|Author (truncated)|Version
---|---|---
vscode-css-formatter|aes|1.0.1
vbscript|Dar|1.0.4
nightswitch-lite|gha|2.2.1
csharp|ms-|1.17.0
vsliveshare|ms-|0.3.897
team|ms-|1.142.0
</details>
<!-- generated by issue reporter --> | feature-request,keybindings,windows | low | Critical |
376,910,694 | rust | Run specific test by fully qualified name | Suppose I have two test targets:
1. tests/tests1.rs
```rust
#[test]
fn test1() {
panic!()
}
#[test]
fn test2() {}
```
2. tests/tests2.rs
```rust
#[test]
fn test1() {}
#[test]
fn test2() {
panic!()
}
```
I want to run only test1 from tests1 and test2 from tests2. The `--exact` option doesn't help me in this case.
I want to have some option to run a specific test, like this:
```
cargo test --no-fail-fast --test tests1 --test tests2 -- --fqn tests1::test1 --fqn tests2::test2
``` | C-enhancement,A-libtest,T-testing-devex | low | Minor |
376,955,034 | TypeScript | Allow setters to return | <!-- π¨ STOP π¨ π¦π§π’π£ π¨ πΊπ»πΆπ· π¨
Half of all issues filed here are duplicates, answered in the FAQ, or not appropriate for the bug tracker. Please read the FAQ first, especially the "Common Feature Requests" section.
-->
## Search Terms
Setters cannot return a value
decorators
<!-- List of keywords you searched for before creating this issue. Write them down here so that others can find this suggestion more easily -->
## Suggestion
By default JavaScript does nothing with the return value of a setter, but it isn't an error.
Allow setters to return as the default behaviour and add flag to turn errors on.
<!-- A summary of what you'd like to see added or changed -->
## Use Cases
- Decorators that override the setter and use the original setters return value.
- Methods that directly call the setter function and expect a return.
<!--
What do you want to use this for?
What shortcomings exist with current approaches?
-->
## Examples
A live example would be [`@computed` from ember-decorators](http://ember-decorators.github.io/ember-decorators/latest/docs/api/modules/@ember-decorators/object#computed), which matches the old behaviour from [ember](https://guides.emberjs.com/release/object-model/computed-properties/#toc_setting-computed-properties).
Code example using legacy decorators (same would be possible with stage 2 decorators):
```ts
function saved (target: {}, key: string | symbol, descriptor: PropertyDescriptor) {
const savedStore = new WeakMap();
const oldSet = descriptor.set;
if (!oldSet) {
throw new Error('Must be used on setter only');
}
const oldGet = descriptor.get;
return {
...descriptor,
get () {
let response = savedStore.get(this);
if (!response && oldGet) {
response = oldGet.call(this);
}
return response;
},
set (value: any) {
const result = oldSet.call(this, value);
savedStore.set(this, result);
}
};
}
class Foo {
@saved
get square () {
// default value of 1 if none is saved
return 1;
}
set square (value: number) {
return value * value;
}
}
```
<!-- Show how this would be used and what the behavior would be -->
## Checklist
My suggestion meets these guidelines:
* [x] This wouldn't be a breaking change in existing TypeScript / JavaScript code
* [x] This wouldn't change the runtime behavior of existing JavaScript code
* [x] This could be implemented without emitting different JS based on the types of the expressions
* [x] This isn't a runtime feature (e.g. new expression-level syntax)
| Suggestion,Awaiting More Feedback | low | Critical |
376,960,106 | rust | Tracking issue for `trait alias` implementation (RFC 1733) | This is the tracking issue for **implementing** (*not* discussing the design) RFC https://github.com/rust-lang/rfcs/pull/1733. It is a subissue of https://github.com/rust-lang/rust/issues/41517.
## Current status
Once #55101 lands, many aspects of trait aliases will be implemented. However, some known limitations remain. These are mostly pre-existing limitations of the trait checker that we intend to lift more generally (see each case below for notes).
**Well-formedness requirements.** We currently require the trait alias to be well-formed. So, for example, `trait Foo<T: Send> { } trait Bar<T> = Foo<T>` is an error. We intend to modify this behavior as part of implementing the implied bounds RFC (https://github.com/rust-lang/rust/issues/44491).
**Trait object associated types.** If you have `trait Foo = Iterator<Item =u32>`, you cannot use the trait object type `dyn Foo`. This is a duplicate of https://github.com/rust-lang/rust/issues/24010.
**Trait object equality.** If you have `trait Foo { }` and `trait Bar = Foo`, we do not currently consider `dyn Foo` and `dyn Bar` to be the same type. Tracking issue https://github.com/rust-lang/rust/issues/55629.
## Pending issues to resolve
- [x] https://github.com/rust-lang/rust/issues/56006 - ICE when used in a library crate
## Deviations and/or clarifications from the RFC
This section is for us to collect notes on deviations from the RFC text, or clarifications to unresolved questions.
## PR history
- [x] https://github.com/rust-lang/rust/pull/45047 by @durka
- [x] https://github.com/rust-lang/rust/pull/55101 by @alexreg
## Other links
- Test scenarios drawn up by @nikomatsakis from the RFC: [gist](https://gist.github.com/nikomatsakis/e4dd2807581fc868ba308382855e68f6) | A-trait-system,B-unstable,B-RFC-implemented,C-tracking-issue,S-tracking-needs-summary,T-types,S-types-deferred | low | Critical |
376,961,544 | rust | trait alias `dyn` type equality doesn't work | If you have `trait Foo { }` and `trait Bar = Foo`, we do not currently consider `dyn Foo` and `dyn Bar` to be the same type.
Subissue of https://github.com/rust-lang/rust/issues/55628 | A-trait-system,T-compiler,C-bug | low | Minor |
376,970,927 | TypeScript | infer-from-usage should infer "truthy" when only undefined is available | ```ts
function f(x, y) {
if (y) {
x = 1
}
return x
}
f(2)
```
**Expected behavior:**
Run infer-from-usage and get `x: number, y?: boolean | undefined`
**Actual behavior:**
`x: number, y?: undefined` | Bug,Domain: Quick Fixes | low | Minor |
376,984,147 | go | cmd/compile: cleanup compiler flag processing | There are (at least) 3 ways in which compiler flags are internally specified and processed. It's a mess. Should clean up and provide a more systematic way of dealing with flags. | Suggested,NeedsFix,compiler/runtime | medium | Critical |
377,002,573 | TypeScript | Can we cut down on `Object.assign` overloads? | [We can convert unions into intersections](https://stackoverflow.com/questions/50374908/transform-union-type-to-intersection-type) (thanks @jcalz!), and we can model variadic args pretty well, so I figured I'd try to model `Object.assign` with a single overload. Here's what I came up with:
```ts
// Maps elements of a tuple to contravariant inference sites.
type MapContravariant<T> = {
[K in keyof T]: (x: T[K]) => void
}
type TupletoIntersection<T, Temp = MapContravariant<T>> =
// Ensure we can index with a number.
Temp extends Record<number, unknown>
// Infer from every element now.
? Temp[number] extends (x: infer U) => unknown ? U : never
: never;
declare function assign<T extends object, Rest extends object[]>(
x: T,
...xs: Rest
): T & TupletoIntersection<Rest>;
```
Unfortunately this doesn't quite give the right results on the following:
```ts
let asdf = assign({x: "hello"}, Math.random() ? {x: "hello"} : {z: true });
```
Currently the type of `asdf` is:
```
| ({ x: string; } & { x: string; z?: undefined; })
| ({ x: string; } & { z: boolean; x?: undefined; })
```
What a beautiful type! Unfortunately you can see that the second element of the union tries to intersect types with conflicting properties for `x` which is nonsense.
Additionally, @weswigham pointed out that this really won't work for the case where you started off with just a plain array whose elements are eventually spread into `Object.assign`.
I do wonder if there's anything better we can do here. | Suggestion,Needs Proposal | low | Major |
377,014,372 | flutter | Keyboard disappears when TextField is dynamically wrapped | ## Steps to Reproduce
1. Run the below code
2. Tap the `TextField`
3. Enter a character
4. Observe that the keyboard immediately disappears and the entered character "disappears" (I expect that the keyboard remains visible and the entered character appears in the `TextField`)
NOTE: I used a `Dismissible` in my repro because that's what I'm using in my real app. However, the problem is still present if I wrap it in something else instead, like a `Container`.
```dart
import 'package:flutter/material.dart';
void main() => runApp(new MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return new MaterialApp(
title: 'Repro',
home: Repro(),
);
}
}
class Repro extends StatefulWidget {
@override
_ReproState createState() => _ReproState();
}
class _ReproState extends State<Repro> {
final Key _textFieldKey = UniqueKey();
final Key _dismissibleKey = UniqueKey();
bool _wrapInDismissible = false;
@override
Widget build(BuildContext context) {
final children = <Widget>[];
final textField = TextField(
key: _textFieldKey,
onChanged: (value) => setState(() => _wrapInDismissible = value != ""),
);
if (!_wrapInDismissible) {
children.add(textField);
} else {
final dismissible = Dismissible(
key: _dismissibleKey,
child: textField,
);
children.add(dismissible);
}
var column = Column(
children: children,
);
var scaffold = Scaffold(appBar: AppBar(title: Text("Repro")),
body: column,);
return scaffold;
}
}
```
## Logs
<details>
<summary> flutter run --verbose</summary>
```
[ +213 ms] executing: [C:\Users\Kent\Repository\flutter\] git rev-parse --abbrev-ref --symbolic @{u}
[ +284 ms] Exit code 0 from: git rev-parse --abbrev-ref --symbolic @{u}
[ ] origin/beta
[ ] executing: [C:\Users\Kent\Repository\flutter\] git rev-parse --abbrev-ref HEAD
[ +127 ms] Exit code 0 from: git rev-parse --abbrev-ref HEAD
[ ] beta
[ +1 ms] executing: [C:\Users\Kent\Repository\flutter\] git ls-remote --get-url origin
[ +115 ms] Exit code 0 from: git ls-remote --get-url origin
[ ] https://github.com/flutter/flutter.git
[ ] executing: [C:\Users\Kent\Repository\flutter\] git log -n 1 --pretty=format:%H
[ +102 ms] Exit code 0 from: git log -n 1 --pretty=format:%H
[ ] f37c235c32fc15babe6dc7b7bc2ee4387e5ecf92
[ ] executing: [C:\Users\Kent\Repository\flutter\] git log -n 1 --pretty=format:%ar
[ +112 ms] Exit code 0 from: git log -n 1 --pretty=format:%ar
[ ] 5 weeks ago
[ +2 ms] executing: [C:\Users\Kent\Repository\flutter\] git describe --match v*.*.* --first-parent --long --tags
[ +113 ms] Exit code 0 from: git describe --match v*.*.* --first-parent --long --tags
[ ] v0.9.4-0-gf37c235c3
[ +533 ms] executing: C:\Users\Kent\AppData\Local\Android\sdk\platform-tools\adb devices -l
[ +162 ms] Exit code 0 from: C:\Users\Kent\AppData\Local\Android\sdk\platform-tools\adb devices -l
[ ] List of devices attached
FA6AF0303979 device product:sailfish model:Pixel device:sailfish transport_id:2
[+1208 ms] C:\Users\Kent\AppData\Local\Android\sdk\platform-tools\adb -s FA6AF0303979 shell getprop
[ +272 ms] ro.hardware = sailfish
[ ] ro.build.characteristics = nosdcard
[+2408 ms] Launching lib/main.dart on Pixel in debug mode...
[ +124 ms] Initializing gradle...
[ +1 ms] Using gradle from C:\Users\Kent\Repository\repro_focus_loss\android\gradlew.bat.
[ +243 ms] executing: C:\Users\Kent\Repository\repro_focus_loss\android\gradlew.bat -v
[+2520 ms]
------------------------------------------------------------
Gradle 4.4
------------------------------------------------------------
Build time: 2017-12-06 09:05:06 UTC
Revision: cf7821a6f79f8e2a598df21780e3ff7ce8db2b82
Groovy: 2.4.12
Ant: Apache Ant(TM) version 1.9.9 compiled on February 2 2017
JVM: 1.8.0_152-release (JetBrains s.r.o 25.152-b02)
OS: Windows 10 10.0 amd64
[ +1 ms] Initializing gradle... (completed)
[ +1 ms] Resolving dependencies...
[ ] executing: [C:\Users\Kent\Repository\repro_focus_loss\android\] C:\Users\Kent\Repository\repro_focus_loss\android\gradlew.bat app:properties
[+5350 ms]
------------------------------------------------------------
Project :app
------------------------------------------------------------
allprojects: [project ':app']
android: com.android.build.gradle.AppExtension_Decorated@2f875164
androidDependencies: task ':app:androidDependencies'
ant: org.gradle.api.internal.project.DefaultAntBuilder@7f788c01
antBuilderFactory: org.gradle.api.internal.project.DefaultAntBuilderFactory@6b6e0ac2
archivesBaseName: app
artifacts: org.gradle.api.internal.artifacts.dsl.DefaultArtifactHandler_Decorated@799e12d5
asDynamicObject: DynamicObject for project ':app'
assemble: task ':app:assemble'
assembleAndroidTest: task ':app:assembleAndroidTest'
assembleDebug: task ':app:assembleDebug'
assembleDebugAndroidTest: task ':app:assembleDebugAndroidTest'
assembleDebugUnitTest: task ':app:assembleDebugUnitTest'
assembleDynamicProfile: task ':app:assembleDynamicProfile'
assembleDynamicProfileUnitTest: task ':app:assembleDynamicProfileUnitTest'
assembleDynamicRelease: task ':app:assembleDynamicRelease'
assembleDynamicReleaseUnitTest: task ':app:assembleDynamicReleaseUnitTest'
assembleProfile: task ':app:assembleProfile'
assembleProfileUnitTest: task ':app:assembleProfileUnitTest'
assembleRelease: task ':app:assembleRelease'
assembleReleaseUnitTest: task ':app:assembleReleaseUnitTest'
baseClassLoaderScope: org.gradle.api.internal.initialization.DefaultClassLoaderScope@6c8760f3
buildDependents: task ':app:buildDependents'
buildDir: C:\Users\Kent\Repository\repro_focus_loss\build\app
buildFile: C:\Users\Kent\Repository\repro_focus_loss\android\app\build.gradle
buildNeeded: task ':app:buildNeeded'
buildOutputs: BaseVariantOutput container
buildPath: :
buildScriptSource: org.gradle.groovy.scripts.TextResourceScriptSource@5724f1e6
buildscript: org.gradle.api.internal.initialization.DefaultScriptHandler@4c87c9da
bundleAppClassesDebug: task ':app:bundleAppClassesDebug'
bundleAppClassesDebugAndroidTest: task ':app:bundleAppClassesDebugAndroidTest'
bundleAppClassesDebugUnitTest: task ':app:bundleAppClassesDebugUnitTest'
bundleAppClassesDynamicProfile: task ':app:bundleAppClassesDynamicProfile'
bundleAppClassesDynamicProfileUnitTest: task ':app:bundleAppClassesDynamicProfileUnitTest'
bundleAppClassesDynamicRelease: task ':app:bundleAppClassesDynamicRelease'
bundleAppClassesDynamicReleaseUnitTest: task ':app:bundleAppClassesDynamicReleaseUnitTest'
bundleAppClassesProfile: task ':app:bundleAppClassesProfile'
bundleAppClassesProfileUnitTest: task ':app:bundleAppClassesProfileUnitTest'
bundleAppClassesRelease: task ':app:bundleAppClassesRelease'
bundleAppClassesReleaseUnitTest: task ':app:bundleAppClassesReleaseUnitTest'
bundleDebugAndroidTestResources: task ':app:bundleDebugAndroidTestResources'
bundleDebugResources: task ':app:bundleDebugResources'
bundleDynamicProfileResources: task ':app:bundleDynamicProfileResources'
bundleDynamicReleaseResources: task ':app:bundleDynamicReleaseResources'
bundleProfileResources: task ':app:bundleProfileResources'
bundleReleaseResources: task ':app:bundleReleaseResources'
check: task ':app:check'
checkDebugManifest: task ':app:checkDebugManifest'
checkDynamicProfileManifest: task ':app:checkDynamicProfileManifest'
checkDynamicReleaseManifest: task ':app:checkDynamicReleaseManifest'
checkProfileManifest: task ':app:checkProfileManifest'
checkReleaseManifest: task ':app:checkReleaseManifest'
childProjects: {}
class: class org.gradle.api.internal.project.DefaultProject_Decorated
classLoaderScope: org.gradle.api.internal.initialization.DefaultClassLoaderScope@45067225
cleanBuildCache: task ':app:cleanBuildCache'
compileDebugAidl: task ':app:compileDebugAidl'
compileDebugAndroidTestAidl: task ':app:compileDebugAndroidTestAidl'
compileDebugAndroidTestJavaWithJavac: task ':app:compileDebugAndroidTestJavaWithJavac'
compileDebugAndroidTestNdk: task ':app:compileDebugAndroidTestNdk'
compileDebugAndroidTestRenderscript: task ':app:compileDebugAndroidTestRenderscript'
compileDebugAndroidTestShaders: task ':app:compileDebugAndroidTestShaders'
compileDebugAndroidTestSources: task ':app:compileDebugAndroidTestSources'
compileDebugJavaWithJavac: task ':app:compileDebugJavaWithJavac'
compileDebugNdk: task ':app:compileDebugNdk'
compileDebugRenderscript: task ':app:compileDebugRenderscript'
compileDebugShaders: task ':app:compileDebugShaders'
compileDebugSources: task ':app:compileDebugSources'
compileDebugUnitTestJavaWithJavac: task ':app:compileDebugUnitTestJavaWithJavac'
compileDebugUnitTestSources: task ':app:compileDebugUnitTestSources'
compileDynamicProfileAidl: task ':app:compileDynamicProfileAidl'
compileDynamicProfileJavaWithJavac: task ':app:compileDynamicProfileJavaWithJavac'
compileDynamicProfileNdk: task ':app:compileDynamicProfileNdk'
compileDynamicProfileRenderscript: task ':app:compileDynamicProfileRenderscript'
compileDynamicProfileShaders: task ':app:compileDynamicProfileShaders'
compileDynamicProfileSources: task ':app:compileDynamicProfileSources'
compileDynamicProfileUnitTestJavaWithJavac: task ':app:compileDynamicProfileUnitTestJavaWithJavac'
compileDynamicProfileUnitTestSources: task ':app:compileDynamicProfileUnitTestSources'
compileDynamicReleaseAidl: task ':app:compileDynamicReleaseAidl'
compileDynamicReleaseJavaWithJavac: task ':app:compileDynamicReleaseJavaWithJavac'
compileDynamicReleaseNdk: task ':app:compileDynamicReleaseNdk'
compileDynamicReleaseRenderscript: task ':app:compileDynamicReleaseRenderscript'
compileDynamicReleaseShaders: task ':app:compileDynamicReleaseShaders'
compileDynamicReleaseSources: task ':app:compileDynamicReleaseSources'
compileDynamicReleaseUnitTestJavaWithJavac: task ':app:compileDynamicReleaseUnitTestJavaWithJavac'
compileDynamicReleaseUnitTestSources: task ':app:compileDynamicReleaseUnitTestSources'
compileLint: task ':app:compileLint'
compileProfileAidl: task ':app:compileProfileAidl'
compileProfileJavaWithJavac: task ':app:compileProfileJavaWithJavac'
compileProfileNdk: task ':app:compileProfileNdk'
compileProfileRenderscript: task ':app:compileProfileRenderscript'
compileProfileShaders: task ':app:compileProfileShaders'
compileProfileSources: task ':app:compileProfileSources'
compileProfileUnitTestJavaWithJavac: task ':app:compileProfileUnitTestJavaWithJavac'
compileProfileUnitTestSources: task ':app:compileProfileUnitTestSources'
compileReleaseAidl: task ':app:compileReleaseAidl'
compileReleaseJavaWithJavac: task ':app:compileReleaseJavaWithJavac'
compileReleaseNdk: task ':app:compileReleaseNdk'
compileReleaseRenderscript: task ':app:compileReleaseRenderscript'
compileReleaseShaders: task ':app:compileReleaseShaders'
compileReleaseSources: task ':app:compileReleaseSources'
compileReleaseUnitTestJavaWithJavac: task ':app:compileReleaseUnitTestJavaWithJavac'
compileReleaseUnitTestSources: task ':app:compileReleaseUnitTestSources'
components: SoftwareComponentInternal set
configurationActions: org.gradle.configuration.project.DefaultProjectConfigurationActionContainer@12d1128e
configurationTargetIdentifier: org.gradle.configuration.ConfigurationTargetIdentifier$1@628a6af3
configurations: configuration container
connectedAndroidTest: task ':app:connectedAndroidTest'
connectedCheck: task ':app:connectedCheck'
connectedDebugAndroidTest: task ':app:connectedDebugAndroidTest'
consumeConfigAttr: task ':app:consumeConfigAttr'
convention: org.gradle.api.internal.plugins.DefaultConvention@1031b661
copyFlutterAssetsDebug: task ':app:copyFlutterAssetsDebug'
copyFlutterAssetsDynamicProfile: task ':app:copyFlutterAssetsDynamicProfile'
copyFlutterAssetsDynamicRelease: task ':app:copyFlutterAssetsDynamicRelease'
copyFlutterAssetsProfile: task ':app:copyFlutterAssetsProfile'
copyFlutterAssetsRelease: task ':app:copyFlutterAssetsRelease'
createDebugCompatibleScreenManifests: task ':app:createDebugCompatibleScreenManifests'
createDynamicProfileCompatibleScreenManifests: task ':app:createDynamicProfileCompatibleScreenManifests'
createDynamicReleaseCompatibleScreenManifests: task ':app:createDynamicReleaseCompatibleScreenManifests'
createProfileCompatibleScreenManifests: task ':app:createProfileCompatibleScreenManifests'
createReleaseCompatibleScreenManifests: task ':app:createReleaseCompatibleScreenManifests'
defaultArtifacts: org.gradle.api.internal.plugins.DefaultArtifactPublicationSet_Decorated@66507918
defaultTasks: []
deferredProjectConfiguration: org.gradle.api.internal.project.DeferredProjectConfiguration@7c0f423e
dependencies: org.gradle.api.internal.artifacts.dsl.dependencies.DefaultDependencyHandler_Decorated@4de372be
depth: 1
description: null
deviceAndroidTest: task ':app:deviceAndroidTest'
deviceCheck: task ':app:deviceCheck'
displayName: project ':app'
distsDir: C:\Users\Kent\Repository\repro_focus_loss\build\app\distributions
distsDirName: distributions
docsDir: C:\Users\Kent\Repository\repro_focus_loss\build\app\docs
docsDirName: docs
ext: org.gradle.api.internal.plugins.DefaultExtraPropertiesExtension@50fc57dd
extensions: org.gradle.api.internal.plugins.DefaultConvention@1031b661
extractProguardFiles: task ':app:extractProguardFiles'
fileOperations: org.gradle.api.internal.file.DefaultFileOperations@35017c63
fileResolver: org.gradle.api.internal.file.BaseDirFileResolver@3399d64f
flutter: FlutterExtension_Decorated@65393400
flutterBuildDebug: task ':app:flutterBuildDebug'
flutterBuildDynamicProfile: task ':app:flutterBuildDynamicProfile'
flutterBuildDynamicRelease: task ':app:flutterBuildDynamicRelease'
flutterBuildProfile: task ':app:flutterBuildProfile'
flutterBuildRelease: task ':app:flutterBuildRelease'
flutterBuildX86Jar: task ':app:flutterBuildX86Jar'
generateDebugAndroidTestAssets: task ':app:generateDebugAndroidTestAssets'
generateDebugAndroidTestBuildConfig: task ':app:generateDebugAndroidTestBuildConfig'
generateDebugAndroidTestResValues: task ':app:generateDebugAndroidTestResValues'
generateDebugAndroidTestResources: task ':app:generateDebugAndroidTestResources'
generateDebugAndroidTestSources: task ':app:generateDebugAndroidTestSources'
generateDebugAssets: task ':app:generateDebugAssets'
generateDebugBuildConfig: task ':app:generateDebugBuildConfig'
generateDebugResValues: task ':app:generateDebugResValues'
generateDebugResources: task ':app:generateDebugResources'
generateDebugSources: task ':app:generateDebugSources'
generateDynamicProfileAssets: task ':app:generateDynamicProfileAssets'
generateDynamicProfileBuildConfig: task ':app:generateDynamicProfileBuildConfig'
generateDynamicProfileResValues: task ':app:generateDynamicProfileResValues'
generateDynamicProfileResources: task ':app:generateDynamicProfileResources'
generateDynamicProfileSources: task ':app:generateDynamicProfileSources'
generateDynamicReleaseAssets: task ':app:generateDynamicReleaseAssets'
generateDynamicReleaseBuildConfig: task ':app:generateDynamicReleaseBuildConfig'
generateDynamicReleaseResValues: task ':app:generateDynamicReleaseResValues'
generateDynamicReleaseResources: task ':app:generateDynamicReleaseResources'
generateDynamicReleaseSources: task ':app:generateDynamicReleaseSources'
generateProfileAssets: task ':app:generateProfileAssets'
generateProfileBuildConfig: task ':app:generateProfileBuildConfig'
generateProfileResValues: task ':app:generateProfileResValues'
generateProfileResources: task ':app:generateProfileResources'
generateProfileSources: task ':app:generateProfileSources'
generateReleaseAssets: task ':app:generateReleaseAssets'
generateReleaseBuildConfig: task ':app:generateReleaseBuildConfig'
generateReleaseResValues: task ':app:generateReleaseResValues'
generateReleaseResources: task ':app:generateReleaseResources'
generateReleaseSources: task ':app:generateReleaseSources'
gradle: build 'android'
group: android
identityPath: :app
inheritedScope: org.gradle.api.internal.ExtensibleDynamicObject$InheritedDynamicObject@57c9c5c5
installDebug: task ':app:installDebug'
installDebugAndroidTest: task ':app:installDebugAndroidTest'
installDynamicProfile: task ':app:installDynamicProfile'
installDynamicRelease: task ':app:installDynamicRelease'
installProfile: task ':app:installProfile'
installRelease: task ':app:installRelease'
javaPreCompileDebug: task ':app:javaPreCompileDebug'
javaPreCompileDebugAndroidTest: task ':app:javaPreCompileDebugAndroidTest'
javaPreCompileDebugUnitTest: task ':app:javaPreCompileDebugUnitTest'
javaPreCompileDynamicProfile: task ':app:javaPreCompileDynamicProfile'
javaPreCompileDynamicProfileUnitTest: task ':app:javaPreCompileDynamicProfileUnitTest'
javaPreCompileDynamicRelease: task ':app:javaPreCompileDynamicRelease'
javaPreCompileDynamicReleaseUnitTest: task ':app:javaPreCompileDynamicReleaseUnitTest'
javaPreCompileProfile: task ':app:javaPreCompileProfile'
javaPreCompileProfileUnitTest: task ':app:javaPreCompileProfileUnitTest'
javaPreCompileRelease: task ':app:javaPreCompileRelease'
javaPreCompileReleaseUnitTest: task ':app:javaPreCompileReleaseUnitTest'
layout: org.gradle.api.internal.file.DefaultProjectLayout@6915593b
libsDir: C:\Users\Kent\Repository\repro_focus_loss\build\app\libs
libsDirName: libs
lint: task ':app:lint'
lintDebug: task ':app:lintDebug'
lintDynamicProfile: task ':app:lintDynamicProfile'
lintDynamicRelease: task ':app:lintDynamicRelease'
lintProfile: task ':app:lintProfile'
lintRelease: task ':app:lintRelease'
lintVitalRelease: task ':app:lintVitalRelease'
logger: org.gradle.internal.logging.slf4j.OutputEventListenerBackedLogger@348759ae
logging: org.gradle.internal.logging.services.DefaultLoggingManager@1443c1f8
mainApkListPersistenceDebug: task ':app:mainApkListPersistenceDebug'
mainApkListPersistenceDebugAndroidTest: task ':app:mainApkListPersistenceDebugAndroidTest'
mainApkListPersistenceDynamicProfile: task ':app:mainApkListPersistenceDynamicProfile'
mainApkListPersistenceDynamicRelease: task ':app:mainApkListPersistenceDynamicRelease'
mainApkListPersistenceProfile: task ':app:mainApkListPersistenceProfile'
mainApkListPersistenceRelease: task ':app:mainApkListPersistenceRelease'
mergeDebugAndroidTestAssets: task ':app:mergeDebugAndroidTestAssets'
mergeDebugAndroidTestJniLibFolders: task ':app:mergeDebugAndroidTestJniLibFolders'
mergeDebugAndroidTestResources: task ':app:mergeDebugAndroidTestResources'
mergeDebugAndroidTestShaders: task ':app:mergeDebugAndroidTestShaders'
mergeDebugAssets: task ':app:mergeDebugAssets'
mergeDebugJniLibFolders: task ':app:mergeDebugJniLibFolders'
mergeDebugResources: task ':app:mergeDebugResources'
mergeDebugShaders: task ':app:mergeDebugShaders'
mergeDynamicProfileAssets: task ':app:mergeDynamicProfileAssets'
mergeDynamicProfileJniLibFolders: task ':app:mergeDynamicProfileJniLibFolders'
mergeDynamicProfileResources: task ':app:mergeDynamicProfileResources'
mergeDynamicProfileShaders: task ':app:mergeDynamicProfileShaders'
mergeDynamicReleaseAssets: task ':app:mergeDynamicReleaseAssets'
mergeDynamicReleaseJniLibFolders: task ':app:mergeDynamicReleaseJniLibFolders'
mergeDynamicReleaseResources: task ':app:mergeDynamicReleaseResources'
mergeDynamicReleaseShaders: task ':app:mergeDynamicReleaseShaders'
mergeProfileAssets: task ':app:mergeProfileAssets'
mergeProfileJniLibFolders: task ':app:mergeProfileJniLibFolders'
mergeProfileResources: task ':app:mergeProfileResources'
mergeProfileShaders: task ':app:mergeProfileShaders'
mergeReleaseAssets: task ':app:mergeReleaseAssets'
mergeReleaseJniLibFolders: task ':app:mergeReleaseJniLibFolders'
mergeReleaseResources: task ':app:mergeReleaseResources'
mergeReleaseShaders: task ':app:mergeReleaseShaders'
mockableAndroidJar: task ':app:mockableAndroidJar'
modelRegistry: org.gradle.model.internal.registry.DefaultModelRegistry@38767e44
modelSchemaStore: org.gradle.model.internal.manage.schema.extract.DefaultModelSchemaStore@378c0e54
module: org.gradle.api.internal.artifacts.ProjectBackedModule@502ec90b
name: app
normalization: org.gradle.normalization.internal.DefaultInputNormalizationHandler_Decorated@21087362
objects: org.gradle.api.internal.model.DefaultObjectFactory@62973af9
org.gradle.jvmargs: -Xmx1536M
packageDebug: task ':app:packageDebug'
packageDebugAndroidTest: task ':app:packageDebugAndroidTest'
packageDynamicProfile: task ':app:packageDynamicProfile'
packageDynamicRelease: task ':app:packageDynamicRelease'
packageProfile: task ':app:packageProfile'
packageRelease: task ':app:packageRelease'
parent: root project 'android'
parentIdentifier: root project 'android'
path: :app
platformAttrExtractor: task ':app:platformAttrExtractor'
pluginManager: org.gradle.api.internal.plugins.DefaultPluginManager_Decorated@68516b52
plugins: [org.gradle.api.plugins.HelpTasksPlugin@15001aaa, com.android.build.gradle.api.AndroidBasePlugin@245215c7, org.gradle.language.base.plugins.LifecycleBasePlugin@3371c2a3, org.gradle.api.plugins.BasePlugin@71cc9c7e, org.gradle.api.plugins.ReportingBasePlugin@54a2f88d, org.gradle.platform.base.plugins.ComponentBasePlugin@5e68b31f, org.gradle.language.base.plugins.LanguageBasePlugin@1dcd4ecf, org.gradle.platform.base.plugins.BinaryBasePlugin@63a2785, org.gradle.api.plugins.JavaBasePlugin@72edb632, com.android.build.gradle.AppPlugin@735abd13, FlutterPlugin@5344a35]
preBuild: task ':app:preBuild'
preDebugAndroidTestBuild: task ':app:preDebugAndroidTestBuild'
preDebugBuild: task ':app:preDebugBuild'
preDebugUnitTestBuild: task ':app:preDebugUnitTestBuild'
preDynamicProfileBuild: task ':app:preDynamicProfileBuild'
preDynamicProfileUnitTestBuild: task ':app:preDynamicProfileUnitTestBuild'
preDynamicReleaseBuild: task ':app:preDynamicReleaseBuild'
preDynamicReleaseUnitTestBuild: task ':app:preDynamicReleaseUnitTestBuild'
preProfileBuild: task ':app:preProfileBuild'
preProfileUnitTestBuild: task ':app:preProfileUnitTestBuild'
preReleaseBuild: task ':app:preReleaseBuild'
preReleaseUnitTestBuild: task ':app:preReleaseUnitTestBuild'
prepareLintJar: task ':app:prepareLintJar'
preparePUBLISHED_DEXDebugAndroidTestForPublishing: task ':app:preparePUBLISHED_DEXDebugAndroidTestForPublishing'
preparePUBLISHED_DEXDebugForPublishing: task ':app:preparePUBLISHED_DEXDebugForPublishing'
preparePUBLISHED_DEXDynamicProfileForPublishing: task ':app:preparePUBLISHED_DEXDynamicProfileForPublishing'
preparePUBLISHED_DEXDynamicReleaseForPublishing: task ':app:preparePUBLISHED_DEXDynamicReleaseForPublishing'
preparePUBLISHED_DEXProfileForPublishing: task ':app:preparePUBLISHED_DEXProfileForPublishing'
preparePUBLISHED_DEXReleaseForPublishing: task ':app:preparePUBLISHED_DEXReleaseForPublishing'
preparePUBLISHED_JAVA_RESDebugAndroidTestForPublishing: task ':app:preparePUBLISHED_JAVA_RESDebugAndroidTestForPublishing'
preparePUBLISHED_JAVA_RESDebugForPublishing: task ':app:preparePUBLISHED_JAVA_RESDebugForPublishing'
preparePUBLISHED_JAVA_RESDynamicProfileForPublishing: task ':app:preparePUBLISHED_JAVA_RESDynamicProfileForPublishing'
preparePUBLISHED_JAVA_RESDynamicReleaseForPublishing: task ':app:preparePUBLISHED_JAVA_RESDynamicReleaseForPublishing'
preparePUBLISHED_JAVA_RESProfileForPublishing: task ':app:preparePUBLISHED_JAVA_RESProfileForPublishing'
preparePUBLISHED_JAVA_RESReleaseForPublishing: task ':app:preparePUBLISHED_JAVA_RESReleaseForPublishing'
preparePUBLISHED_NATIVE_LIBSDebugAndroidTestForPublishing: task ':app:preparePUBLISHED_NATIVE_LIBSDebugAndroidTestForPublishing'
preparePUBLISHED_NATIVE_LIBSDebugForPublishing: task ':app:preparePUBLISHED_NATIVE_LIBSDebugForPublishing'
preparePUBLISHED_NATIVE_LIBSDynamicProfileForPublishing: task ':app:preparePUBLISHED_NATIVE_LIBSDynamicProfileForPublishing'
preparePUBLISHED_NATIVE_LIBSDynamicReleaseForPublishing: task ':app:preparePUBLISHED_NATIVE_LIBSDynamicReleaseForPublishing'
preparePUBLISHED_NATIVE_LIBSProfileForPublishing: task ':app:preparePUBLISHED_NATIVE_LIBSProfileForPublishing'
preparePUBLISHED_NATIVE_LIBSReleaseForPublishing: task ':app:preparePUBLISHED_NATIVE_LIBSReleaseForPublishing'
processDebugAndroidTestJavaRes: task ':app:processDebugAndroidTestJavaRes'
processDebugAndroidTestManifest: task ':app:processDebugAndroidTestManifest'
processDebugAndroidTestResources: task ':app:processDebugAndroidTestResources'
processDebugJavaRes: task ':app:processDebugJavaRes'
processDebugManifest: task ':app:processDebugManifest'
processDebugResources: task ':app:processDebugResources'
processDebugUnitTestJavaRes: task ':app:processDebugUnitTestJavaRes'
processDynamicProfileJavaRes: task ':app:processDynamicProfileJavaRes'
processDynamicProfileManifest: task ':app:processDynamicProfileManifest'
processDynamicProfileResources: task ':app:processDynamicProfileResources'
processDynamicProfileUnitTestJavaRes: task ':app:processDynamicProfileUnitTestJavaRes'
processDynamicReleaseJavaRes: task ':app:processDynamicReleaseJavaRes'
processDynamicReleaseManifest: task ':app:processDynamicReleaseManifest'
processDynamicReleaseResources: task ':app:processDynamicReleaseResources'
processDynamicReleaseUnitTestJavaRes: task ':app:processDynamicReleaseUnitTestJavaRes'
processOperations: org.gradle.api.internal.file.DefaultFileOperations@35017c63
processProfileJavaRes: task ':app:processProfileJavaRes'
processProfileManifest: task ':app:processProfileManifest'
processProfileResources: task ':app:processProfileResources'
processProfileUnitTestJavaRes: task ':app:processProfileUnitTestJavaRes'
processReleaseJavaRes: task ':app:processReleaseJavaRes'
processReleaseManifest: task ':app:processReleaseManifest'
processReleaseResources: task ':app:processReleaseResources'
processReleaseUnitTestJavaRes: task ':app:processReleaseUnitTestJavaRes'
project: project ':app'
projectConfigurator: org.gradle.api.internal.project.BuildOperationCrossProjectConfigurator@635ebc42
projectDir: C:\Users\Kent\Repository\repro_focus_loss\android\app
projectEvaluationBroadcaster: ProjectEvaluationListener broadcast
projectEvaluator: org.gradle.configuration.project.LifecycleProjectEvaluator@12f5028
projectPath: :app
projectRegistry: org.gradle.api.internal.project.DefaultProjectRegistry@5a34e206
properties: {...}
providers: org.gradle.api.internal.provider.DefaultProviderFactory@9876e1d
reportBuildArtifactsDebug: task ':app:reportBuildArtifactsDebug'
reportBuildArtifactsDynamicProfile: task ':app:reportBuildArtifactsDynamicProfile'
reportBuildArtifactsDynamicRelease: task ':app:reportBuildArtifactsDynamicRelease'
reportBuildArtifactsProfile: task ':app:reportBuildArtifactsProfile'
reportBuildArtifactsRelease: task ':app:reportBuildArtifactsRelease'
reporting: org.gradle.api.reporting.ReportingExtension_Decorated@39dc19e9
reportsDir: C:\Users\Kent\Repository\repro_focus_loss\build\app\reports
repositories: repository container
resolveConfigAttr: task ':app:resolveConfigAttr'
resourceLoader: org.gradle.internal.resource.transfer.DefaultUriTextResourceLoader@2f761e3c
resources: org.gradle.api.internal.resources.DefaultResourceHandler@54a3b5ea
rootDir: C:\Users\Kent\Repository\repro_focus_loss\android
rootProject: root project 'android'
script: false
scriptHandlerFactory: org.gradle.api.internal.initialization.DefaultScriptHandlerFactory@297a59aa
scriptPluginFactory: org.gradle.configuration.ScriptPluginFactorySelector@70301e3d
serviceRegistryFactory: org.gradle.internal.service.scopes.ProjectScopeServices$4@6fa869f9
services: ProjectScopeServices
signingReport: task ':app:signingReport'
sourceCompatibility: 1.8
sourceSets: SourceSet container
splitsDiscoveryTaskDebug: task ':app:splitsDiscoveryTaskDebug'
splitsDiscoveryTaskDynamicProfile: task ':app:splitsDiscoveryTaskDynamicProfile'
splitsDiscoveryTaskDynamicRelease: task ':app:splitsDiscoveryTaskDynamicRelease'
splitsDiscoveryTaskProfile: task ':app:splitsDiscoveryTaskProfile'
splitsDiscoveryTaskRelease: task ':app:splitsDiscoveryTaskRelease'
standardOutputCapture: org.gradle.internal.logging.services.DefaultLoggingManager@1443c1f8
state: project state 'EXECUTED'
status: integration
subprojects: []
targetCompatibility: 1.8
tasks: task set
test: task ':app:test'
testDebugUnitTest: task ':app:testDebugUnitTest'
testDynamicProfileUnitTest: task ':app:testDynamicProfileUnitTest'
testDynamicReleaseUnitTest: task ':app:testDynamicReleaseUnitTest'
testProfileUnitTest: task ':app:testProfileUnitTest'
testReleaseUnitTest: task ':app:testReleaseUnitTest'
testReportDir: C:\Users\Kent\Repository\repro_focus_loss\build\app\reports\tests
testReportDirName: tests
testResultsDir: C:\Users\Kent\Repository\repro_focus_loss\build\app\test-results
testResultsDirName: test-results
transformClassesWithDexBuilderForDebug: task ':app:transformClassesWithDexBuilderForDebug'
transformClassesWithDexBuilderForDebugAndroidTest: task ':app:transformClassesWithDexBuilderForDebugAndroidTest'
transformClassesWithDexBuilderForDynamicProfile: task ':app:transformClassesWithDexBuilderForDynamicProfile'
transformClassesWithDexBuilderForDynamicRelease: task ':app:transformClassesWithDexBuilderForDynamicRelease'
transformClassesWithDexBuilderForProfile: task ':app:transformClassesWithDexBuilderForProfile'
transformClassesWithDexBuilderForRelease: task ':app:transformClassesWithDexBuilderForRelease'
transformDexArchiveWithDexMergerForDebug: task ':app:transformDexArchiveWithDexMergerForDebug'
transformDexArchiveWithDexMergerForDebugAndroidTest: task ':app:transformDexArchiveWithDexMergerForDebugAndroidTest'
transformDexArchiveWithDexMergerForDynamicProfile: task ':app:transformDexArchiveWithDexMergerForDynamicProfile'
transformDexArchiveWithDexMergerForDynamicRelease: task ':app:transformDexArchiveWithDexMergerForDynamicRelease'
transformDexArchiveWithDexMergerForProfile: task ':app:transformDexArchiveWithDexMergerForProfile'
transformDexArchiveWithDexMergerForRelease: task ':app:transformDexArchiveWithDexMergerForRelease'
transformDexArchiveWithExternalLibsDexMergerForDebug: task ':app:transformDexArchiveWithExternalLibsDexMergerForDebug'
transformDexArchiveWithExternalLibsDexMergerForDebugAndroidTest: task ':app:transformDexArchiveWithExternalLibsDexMergerForDebugAndroidTest'
transformDexArchiveWithExternalLibsDexMergerForDynamicProfile: task ':app:transformDexArchiveWithExternalLibsDexMergerForDynamicProfile'
transformDexArchiveWithExternalLibsDexMergerForDynamicRelease: task ':app:transformDexArchiveWithExternalLibsDexMergerForDynamicRelease'
transformDexArchiveWithExternalLibsDexMergerForProfile: task ':app:transformDexArchiveWithExternalLibsDexMergerForProfile'
transformDexArchiveWithExternalLibsDexMergerForRelease: task ':app:transformDexArchiveWithExternalLibsDexMergerForRelease'
transformNativeLibsWithMergeJniLibsForDebug: task ':app:transformNativeLibsWithMergeJniLibsForDebug'
transformNativeLibsWithMergeJniLibsForDebugAndroidTest: task ':app:transformNativeLibsWithMergeJniLibsForDebugAndroidTest'
transformNativeLibsWithMergeJniLibsForDynamicProfile: task ':app:transformNativeLibsWithMergeJniLibsForDynamicProfile'
transformNativeLibsWithMergeJniLibsForDynamicRelease: task ':app:transformNativeLibsWithMergeJniLibsForDynamicRelease'
transformNativeLibsWithMergeJniLibsForProfile: task ':app:transformNativeLibsWithMergeJniLibsForProfile'
transformNativeLibsWithMergeJniLibsForRelease: task ':app:transformNativeLibsWithMergeJniLibsForRelease'
transformResourcesWithMergeJavaResForDebug: task ':app:transformResourcesWithMergeJavaResForDebug'
transformResourcesWithMergeJavaResForDebugAndroidTest: task ':app:transformResourcesWithMergeJavaResForDebugAndroidTest'
transformResourcesWithMergeJavaResForDebugUnitTest: task ':app:transformResourcesWithMergeJavaResForDebugUnitTest'
transformResourcesWithMergeJavaResForDynamicProfile: task ':app:transformResourcesWithMergeJavaResForDynamicProfile'
transformResourcesWithMergeJavaResForDynamicProfileUnitTest: task ':app:transformResourcesWithMergeJavaResForDynamicProfileUnitTest'
transformResourcesWithMergeJavaResForDynamicRelease: task ':app:transformResourcesWithMergeJavaResForDynamicRelease'
transformResourcesWithMergeJavaResForDynamicReleaseUnitTest: task ':app:transformResourcesWithMergeJavaResForDynamicReleaseUnitTest'
transformResourcesWithMergeJavaResForProfile: task ':app:transformResourcesWithMergeJavaResForProfile'
transformResourcesWithMergeJavaResForProfileUnitTest: task ':app:transformResourcesWithMergeJavaResForProfileUnitTest'
transformResourcesWithMergeJavaResForRelease: task ':app:transformResourcesWithMergeJavaResForRelease'
transformResourcesWithMergeJavaResForReleaseUnitTest: task ':app:transformResourcesWithMergeJavaResForReleaseUnitTest'
uninstallAll: task ':app:uninstallAll'
uninstallDebug: task ':app:uninstallDebug'
uninstallDebugAndroidTest: task ':app:uninstallDebugAndroidTest'
uninstallDynamicProfile: task ':app:uninstallDynamicProfile'
uninstallDynamicRelease: task ':app:uninstallDynamicRelease'
uninstallProfile: task ':app:uninstallProfile'
uninstallRelease: task ':app:uninstallRelease'
validateSigningDebug: task ':app:validateSigningDebug'
validateSigningDebugAndroidTest: task ':app:validateSigningDebugAndroidTest'
validateSigningDynamicProfile: task ':app:validateSigningDynamicProfile'
validateSigningDynamicRelease: task ':app:validateSigningDynamicRelease'
validateSigningProfile: task ':app:validateSigningProfile'
validateSigningRelease: task ':app:validateSigningRelease'
version: unspecified
writeDebugApplicationId: task ':app:writeDebugApplicationId'
writeDynamicProfileApplicationId: task ':app:writeDynamicProfileApplicationId'
writeDynamicReleaseApplicationId: task ':app:writeDynamicReleaseApplicationId'
writeProfileApplicationId: task ':app:writeProfileApplicationId'
writeReleaseApplicationId: task ':app:writeReleaseApplicationId'
1 actionable task: 1 executed
[ +68 ms] Resolving dependencies... (completed)
[ +6 ms] executing: C:\Users\Kent\AppData\Local\Android\sdk\build-tools\28.0.2\aapt dump xmltree C:\Users\Kent\Repository\repro_focus_loss\build\app\outputs\apk\app.apk AndroidManifest.xml
[ +157 ms] Exit code 0 from: C:\Users\Kent\AppData\Local\Android\sdk\build-tools\28.0.2\aapt dump xmltree C:\Users\Kent\Repository\repro_focus_loss\build\app\outputs\apk\app.apk AndroidManifest.xml
[ ] N: android=http://schemas.android.com/apk/res/android
E: manifest (line=2)
A: android:versionCode(0x0101021b)=(type 0x10)0x1
A: android:versionName(0x0101021c)="1.0.0" (Raw: "1.0.0")
A: package="com.example.reprofocusloss" (Raw: "com.example.reprofocusloss")
E: uses-sdk (line=7)
A: android:minSdkVersion(0x0101020c)=(type 0x10)0x10
A: android:targetSdkVersion(0x01010270)=(type 0x10)0x1b
E: uses-permission (line=16)
A: android:name(0x01010003)="android.permission.INTERNET" (Raw: "android.permission.INTERNET")
E: application (line=24)
A: android:label(0x01010001)="repro_focus_loss" (Raw: "repro_focus_loss")
A: android:icon(0x01010002)=@0x7f020000
A: android:name(0x01010003)="io.flutter.app.FlutterApplication" (Raw: "io.flutter.app.FlutterApplication")
A: android:debuggable(0x0101000f)=(type 0x12)0xffffffff
E: activity (line=29)
A: android:theme(0x01010000)=@0x7f030000
A: android:name(0x01010003)="com.example.reprofocusloss.MainActivity" (Raw: "com.example.reprofocusloss.MainActivity")
A: android:launchMode(0x0101001d)=(type 0x10)0x1
A: android:configChanges(0x0101001f)=(type 0x11)0x400035b4
A: android:windowSoftInputMode(0x0101022b)=(type 0x11)0x10
A: android:hardwareAccelerated(0x010102d3)=(type 0x12)0xffffffff
E: meta-data (line=43)
A: android:name(0x01010003)="io.flutter.app.android.SplashScreenUntilFirstFrame" (Raw: "io.flutter.app.android.SplashScreenUntilFirstFrame")
A: android:value(0x01010024)=(type 0x12)0xffffffff
E: intent-filter (line=47)
E: action (line=48)
A: android:name(0x01010003)="android.intent.action.MAIN" (Raw: "android.intent.action.MAIN")
E: category (line=50)
A: android:name(0x01010003)="android.intent.category.LAUNCHER" (Raw: "android.intent.category.LAUNCHER")
[ +33 ms] executing: C:\Users\Kent\AppData\Local\Android\sdk\platform-tools\adb -s FA6AF0303979 shell -x logcat -v time -t 1
[ +314 ms] Exit code 0 from: C:\Users\Kent\AppData\Local\Android\sdk\platform-tools\adb -s FA6AF0303979 shell -x logcat -v time -t 1
[ ] --------- beginning of main
11-03 12:10:25.193 I/EventLogSendingHelper(30221): Sending log events.
[ +10 ms] executing: C:\Users\Kent\AppData\Local\Android\sdk\platform-tools\adb -s FA6AF0303979 shell -x logcat -v time
[+1318 ms] DependencyChecker: nothing is modified after 2018-11-03 12:08:51.000.
[ +13 ms] executing: C:\Users\Kent\AppData\Local\Android\sdk\platform-tools\adb version
[ +207 ms] Android Debug Bridge version 1.0.40
Version 4986621
Installed as C:\Users\Kent\AppData\Local\Android\sdk\platform-tools\adb.EXE
[ +8 ms] executing: C:\Users\Kent\AppData\Local\Android\sdk\platform-tools\adb start-server
[ +143 ms] Building APK
[ +54 ms] Gradle task 'assembleDebug'...
[ +7 ms] executing: [C:\Users\Kent\Repository\repro_focus_loss\android\] C:\Users\Kent\Repository\repro_focus_loss\android\gradlew.bat -Pverbose=true -Ptarget=C:\Users\Kent\Repository\repro_focus_loss\lib/main.dart -Ptarget-platform=android-arm64 assembleDebug
[+5680 ms] 30 actionable tasks: 3 executed, 27 up-to-date
[ +483 ms] Gradle task 'assembleDebug'... (completed)
[ +63 ms] calculateSha: LocalDirectory: 'C:\Users\Kent\Repository\repro_focus_loss\build\app\outputs\apk'/app.apk
[+1113 ms] Built build\app\outputs\apk\debug\app-debug.apk.
[ +1 ms] executing: C:\Users\Kent\AppData\Local\Android\sdk\build-tools\28.0.2\aapt dump xmltree C:\Users\Kent\Repository\repro_focus_loss\build\app\outputs\apk\app.apk AndroidManifest.xml
[ +82 ms] Exit code 0 from: C:\Users\Kent\AppData\Local\Android\sdk\build-tools\28.0.2\aapt dump xmltree C:\Users\Kent\Repository\repro_focus_loss\build\app\outputs\apk\app.apk AndroidManifest.xml
[ ] N: android=http://schemas.android.com/apk/res/android
E: manifest (line=2)
A: android:versionCode(0x0101021b)=(type 0x10)0x1
A: android:versionName(0x0101021c)="1.0.0" (Raw: "1.0.0")
A: package="com.example.reprofocusloss" (Raw: "com.example.reprofocusloss")
E: uses-sdk (line=7)
A: android:minSdkVersion(0x0101020c)=(type 0x10)0x10
A: android:targetSdkVersion(0x01010270)=(type 0x10)0x1b
E: uses-permission (line=16)
A: android:name(0x01010003)="android.permission.INTERNET" (Raw: "android.permission.INTERNET")
E: application (line=24)
A: android:label(0x01010001)="repro_focus_loss" (Raw: "repro_focus_loss")
A: android:icon(0x01010002)=@0x7f020000
A: android:name(0x01010003)="io.flutter.app.FlutterApplication" (Raw: "io.flutter.app.FlutterApplication")
A: android:debuggable(0x0101000f)=(type 0x12)0xffffffff
E: activity (line=29)
A: android:theme(0x01010000)=@0x7f030000
A: android:name(0x01010003)="com.example.reprofocusloss.MainActivity" (Raw: "com.example.reprofocusloss.MainActivity")
A: android:launchMode(0x0101001d)=(type 0x10)0x1
A: android:configChanges(0x0101001f)=(type 0x11)0x400035b4
A: android:windowSoftInputMode(0x0101022b)=(type 0x11)0x10
A: android:hardwareAccelerated(0x010102d3)=(type 0x12)0xffffffff
E: meta-data (line=43)
A: android:name(0x01010003)="io.flutter.app.android.SplashScreenUntilFirstFrame" (Raw: "io.flutter.app.android.SplashScreenUntilFirstFrame")
A: android:value(0x01010024)=(type 0x12)0xffffffff
E: intent-filter (line=47)
E: action (line=48)
A: android:name(0x01010003)="android.intent.action.MAIN" (Raw: "android.intent.action.MAIN")
E: category (line=50)
A: android:name(0x01010003)="android.intent.category.LAUNCHER" (Raw: "android.intent.category.LAUNCHER")
[ ] Stopping app 'app.apk' on Pixel.
[ ] executing: C:\Users\Kent\AppData\Local\Android\sdk\platform-tools\adb -s FA6AF0303979 shell am force-stop com.example.reprofocusloss
[ +279 ms] executing: C:\Users\Kent\AppData\Local\Android\sdk\platform-tools\adb -s FA6AF0303979 shell pm list packages com.example.reprofocusloss
[ +315 ms] package:com.example.reprofocusloss
[ +8 ms] executing: C:\Users\Kent\AppData\Local\Android\sdk\platform-tools\adb -s FA6AF0303979 shell cat /data/local/tmp/sky.com.example.reprofocusloss.sha1
[ +248 ms] dca07884682690791311801d24959a6236b642d0
[ +3 ms] Latest build already installed.
[ ] Pixel startApp
[ +4 ms] executing: C:\Users\Kent\AppData\Local\Android\sdk\platform-tools\adb -s FA6AF0303979 shell am start -a android.intent.action.RUN -f 0x20000000 --ez enable-background-compilation true --ez enable-dart-profiling true --ez enable-checked-mode true com.example.reprofocusloss/com.example.reprofocusloss.MainActivity
[ +325 ms] Starting: Intent { act=android.intent.action.RUN flg=0x20000000 cmp=com.example.reprofocusloss/.MainActivity (has extras) }
[ ] Waiting for observatory port to be available...
[ +533 ms] Observatory URL on device: http://127.0.0.1:44993/
[ +2 ms] executing: C:\Users\Kent\AppData\Local\Android\sdk\platform-tools\adb -s FA6AF0303979 forward tcp:0 tcp:44993
[ +78 ms] 59124
[ ] Forwarded host port 59124 to device port 44993 for Observatory
[ +11 ms] Connecting to service protocol: http://127.0.0.1:59124/
[ +461 ms] Successfully connected to service protocol: http://127.0.0.1:59124/
[ +6 ms] getVM: {}
[ +25 ms] getIsolate: {isolateId: isolates/467938155}
[ +7 ms] _flutter.listViews: {isolateId: isolates/467938155}
[ +84 ms] DevFS: Creating new filesystem on the device (null)
[ +1 ms] _createDevFS: {fsName: repro_focus_loss}
[ +58 ms] DevFS: Created new filesystem on the device (file:///data/user/0/com.example.reprofocusloss/cache/repro_focus_lossACKURS/repro_focus_loss/)
[ +5 ms] Updating assets
[ +402 ms] Syncing files to device Pixel...
[ +5 ms] DevFS: Starting sync from LocalDirectory: 'C:\Users\Kent\Repository\repro_focus_loss'
[ ] Scanning project files
[ +8 ms] Scanning package files
[ +137 ms] Scanning asset files
[ +1 ms] Scanning for deleted files
[ +73 ms] Compiling dart to kernel with 436 updated files
[ +21 ms] C:\Users\Kent\Repository\flutter\bin\cache\dart-sdk\bin\dart C:\Users\Kent\Repository\flutter\bin\cache\artifacts\engine\windows-x64\frontend_server.dart.snapshot --sdk-root C:\Users\Kent\Repository\flutter\bin\cache\artifacts\engine\common\flutter_patched_sdk/ --incremental --strong --target=flutter --output-dill build\app.dill --packages C:\Users\Kent\Repository\repro_focus_loss\.packages --filesystem-scheme org-dartlang-root
[+4532 ms] Updating files
[ +254 ms] DevFS: Sync finished
[ ] Syncing files to device Pixel... (completed)
[ +2 ms] Synced 0.8MB.
[ +7 ms] _flutter.listViews: {isolateId: isolates/467938155}
[ +25 ms] Connected to _flutterView/0x73a8e15f98.
[ +10 ms] β‘ΖΓΆΓ To hot reload changes while running, press "r". To hot restart (and rebuild state), press "R".
[ +3 ms] An Observatory debugger and profiler on Pixel is available at: http://127.0.0.1:59124/
[ ] For a more detailed help message, press "h". To detach, press "d"; to quit, press "q".
```
### flutter analyze
```
Analyzing repro_focus_loss...
No issues found! (ran in 6.2s)
```
### flutter doctor
```
Doctor summary (to see all details, run flutter doctor -v):
[β] Flutter (Channel beta, v0.9.4, on Microsoft Windows [Version 10.0.17134.345], locale en-AU)
[β] Android toolchain - develop for Android devices (Android SDK 28.0.2)
[β] Android Studio (version 3.1)
X Flutter plugin not installed; this adds Flutter specific functionality.
X Dart plugin not installed; this adds Dart specific functionality.
[!] VS Code (version 1.28.2)
[β] Connected devices (1 available)
! Doctor found issues in 1 category.
```
</details> | a: text input,framework,has reproducible steps,P3,found in release: 3.10,found in release: 3.12,team-framework,triaged-framework | low | Critical |
377,023,370 | terminal | Problems with font "zooming" | The first problem is that there's no way to reset it to "100%" -- Frankly, my suggestion is that you should support hotkeys like browsers do:
- `{Ctrl}+{=}` to zoom in
- `{Ctrl}+{-}` to zoom out
- `{Ctrl}+{0}` to reset it to normal...
The second problem is that zooming resizes the window -- which is particularly frustrating when scrolling sends parts of the window off-screen, even though I started out _snapped_ to an edge or corner.
When the window is snapped to the screen edges, the system sizes the window to the screen size, rather than a multiple of the CHARACTER size -- so if the font isn't exactly the perfect size for that, it's forced. The problem is that if I zoom in, and zoom back out ... it doesn't go back to the _original size_, but instead to the nearest size based on the font!
So if I start out at this size (note the awkward space between the blue timestamp and the scroll bar due to snapping the window):

When I zoom in and zoom back out (or vice-versa) it ends up with that awkward space on the _outside_ of the window:

Recorded in action: https://i.imgur.com/nux7AqY.gifv
I *hoped* that unchecking the "Let system position window" in the layout would stop it from doing that, but no such luck. Can we get an extra option in there to control whether zooming resizes the window, or changes the number of characters in the window instead? | Product-Conhost,Area-Interaction,Issue-Bug | low | Major |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.