licenses
sequencelengths 1
3
| version
stringclasses 677
values | tree_hash
stringlengths 40
40
| path
stringclasses 1
value | type
stringclasses 2
values | size
stringlengths 2
8
| text
stringlengths 25
67.1M
| package_name
stringlengths 2
41
| repo
stringlengths 33
86
|
---|---|---|---|---|---|---|---|---|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 3397 |
"""
function chain(X, arr::Array{L,1}) where {L<:Layer}
Returns the input `Layer` and the output `Layer` from an `Array` of layers and the input of the model as and `Array` `X`
"""
function chain(X, arr::Array{L,1}) where {L<:Layer}
if ! (arr[1] isa Input)
X_Input = Input(X)
end
for l=1:length(arr)
if l==1
if ! isa(arr[l],Input)
X = arr[l](X_Input)
else
X_Input = X = arr[l](X)
end
else
X = arr[l](X)
end
end #for
return X_Input, X
end
export chain
"""
connect with the previous layer
"""
function (l::FCLayer)(li_1::Layer)
l.prevLayer = li_1
if ! in(l,li_1.nextLayers)
push!(li_1.nextLayers, l)
end
l.inputS = li_1.outputS
l.outputS = (l.channels, li_1.outputS[2])
return l
end #function (l::FCLayer)(li_1::Layer)
function (l::Activation)(li_1::Layer)
l.prevLayer = li_1
l.channels = li_1.channels
l.inputS = l.outputS = li_1.outputS
if ! in(l,li_1.nextLayers)
push!(li_1.nextLayers, l)
end
return l
end
function (l::Flatten)(li_1::Layer)
l.prevLayer = li_1
l.inputS = li_1.outputS
l.channels = prod(l.inputS[1:end-1])
l.outputS = (l.channels, l.inputS[end])
if !(l in li_1.nextLayers)
push!(li_1.nextLayers, l)
end
return l
end
function (l::BatchNorm)(li_1::Layer)
l.prevLayer = li_1
l.channels = li_1.channels
N = length(li_1.outputS)
if l.dim >= N
throw(DimensionMismatch("Normalization Dimension must be less than $N"))
end
l.inputS = l.outputS = li_1.outputS
if ! in(l,li_1.nextLayers)
push!(li_1.nextLayers, l)
end
return l
end #function (l::BatchNorm)
function (l::ConcatLayer)(ls::Array{L,1}) where {L<:Layer}
for li in ls
if !in(li,l.prevLayer)
push!(l.prevLayer, li)
end
if !in(l, li.nextLayers)
push!(li.nextLayers, l)
end
end #for
l.inputS = ls[1].outputS
l.channels = sum(li.channels for li in ls)
l.LSlice[l.prevLayer[1]] = 1:l.prevLayer[1].channels
for i=2:length(l.prevLayer)
l.LSlice[l.prevLayer[i]] = (l.prevLayer[i-1].channels+1) : (l.prevLayer[i-1].channels+l.prevLayer[i].channels)
end
l.outputS = (l.inputS[1:end-2]...,l.channels,l.inputS[end])
return l
end #function (l::AddLayer)(li::Array{Layer,1})
function (l::AddLayer)(ls::Array{L,1}) where {L<:Layer}
for li in ls
if !in(li,l.prevLayer)
push!(l.prevLayer, li)
end
if !in(l, li.nextLayers)
push!(li.nextLayers, l)
end
end #for
l.inputS = l.outputS = ls[1].outputS
l.channels = ls[1].channels
return l
end #function (l::AddLayer)(li::Array{Layer,1})
"""
define input as X
"""
function (l::FCLayer)(x::Array)
l.prevLayer = nothing
return l
end #function (l::FCLayer)(x::Array)
function (l::Conv1D)(x::Array)
l.prevLayer = nothing
return l
end
function (l::Conv2D)(x::Array)
l.prevLayer = nothing
return l
end
function (l::Conv3D)(x::Array)
l.prevLayer = nothing
return l
end
function (l::Input)(X::AbstractArray{T,N}) where {T,N}
l.A = X
channels = size(X)[end-1]
l.channels = channels
return l
end #function (l::Input)(X::AbstractArray{T,N})
export l
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 11235 |
"""
flatten 2D matrix into (m*n, 1) matrix
mainly used for images to flatten images
inputs:
x := 3D (rgp, m, n) matrix
outputs:
y := 2D (rgp*m*n, 1) matrix
"""
function flatten(x)
rgp, n, m = size(x)
return reshape(x, (rgp*m*n, 1))
end
"""
perform the forward propagation using
input:
x := (n0, m) matrix
y := (c, m) matrix where c is the number of classes
return cache of A, Z, Yhat, Cost
"""
function forwardProp(X::Matrix{T},
Y::Matrix{T},
model::Model) where {T}
W::AbstractArray{Matrix{T},1},
B::AbstractArray{Matrix{T},1},
layers::AbstractArray{Layer,1},
lossFun = model.W, model.B, model.layers, model.lossFun
regulization = model.regulization
λ = model.λ
m = size(X)[2]
c = size(Y)[1]
L = length(layers)
A = Vector{Matrix{eltype(X)}}()
Z = Vector{Matrix{eltype(X)}}()
push!(Z, W[1]*X .+ B[1])
actFun = layers[1].actFun
push!(A, eval(:($actFun.($Z[1]))))
for l=2:L
push!(Z, W[l]*A[l-1] .+ B[l])
actFun = layers[l].actFun
if isequal(actFun, :softmax)
a = Matrix{eltype(X)}(undef, c, 0)
for i=1:m
zCol = Z[l][:,i]
a = hcat(a, eval(:($(actFun)($zCol))))
end
push!(A, a)
else
push!(A, eval(:($(actFun).($Z[$l]))))
end
end
if isequal(lossFun, :categoricalCrossentropy)
cost = sum(eval(:($lossFun.($A[$L], $Y))))/ m
else
cost = sum(eval(:($lossFun.($A[$L], $Y)))) / (m*c)
end
if regulization > 0
cost += (λ/2m) * sum([norm(w, regulization) for w in W])
end
return Dict("A"=>A,
"Z"=>Z,
"Yhat"=>A[L],
"Cost"=>cost)
end #forwardProp
# export forwardProp
"""
predict Y using the model and the input X and the labels Y
inputs:
model := the trained model
X := the input matrix
Y := the input labels to compare with
output:
a Dict of
"Yhat" := the predicted values
"Yhat_bools" := the predicted labels
"accuracy" := the accuracy of the predicted labels
"""
function predict(model::Model, X, Y)
Ŷ = forwardProp(X, Y, model)["Yhat"]
T = eltype(Ŷ)
layers = model.layers
c, m = size(Y)
# if isbool(Y)
acc = 0
if isequal(layers[end].actFun, :softmax)
Ŷ_bool = BitArray(undef, c, 0)
p = Progress(size(Ŷ)[2], 0.01)
for v in eachcol(Ŷ)
Ŷ_bool = hcat(Ŷ_bool, v .== maximum(v))
next!(p)
end
acc = sum([Ŷ_bool[:,i] == Y[:,i] for i=1:size(Y)[2]])/m
println("Accuracy is = $acc")
end
if isequal(layers[end].actFun, :σ)
Ŷ_bool = BitArray(undef, c, 0)
p = Progress(size(Ŷ)[2], 0.01)
for v in eachcol(Ŷ)
Ŷ_bool = hcat(Ŷ_bool, v .> T(0.5))
next!(p)
end
acc = sum(Ŷ_bool .== Y)/(c*m)
println("Accuracy is = $acc")
end
return Dict("Yhat"=>Ŷ,
"Yhat_bool"=>Ŷ_bool,
"accuracy"=>acc)
end #predict
function backProp(X,Y,
model::Model,
cache::Dict{})
layers::AbstractArray{Layer, 1} = model.layers
lossFun = model.lossFun
m = size(X)[2]
L = length(layers)
A, Z = cache["A"], cache["Z"]
W, B, regulization, λ = model.W, model.B, model.regulization, model.λ
D = [rand(size(A[i])...) .< layers[i].keepProb for i=1:L]
if layers[L].keepProb < 1.0 #to save time of multiplication in case keepProb was one
A = [A[i] .* D[i] for i=1:L]
A = [A[i] ./ layers[i].keepProb for i=1:L]
end
# init all Arrays
dA = Vector{Matrix{eltype(A[1])}}([similar(mat) for mat in A])
dZ = Vector{Matrix{eltype(Z[1])}}([similar(mat) for mat in Z])
dW = Vector{Matrix{eltype(W[1])}}([similar(mat) for mat in W])
dB = Vector{Matrix{eltype(B[1])}}([similar(mat) for mat in B])
dlossFun = Symbol("d",lossFun)
actFun = layers[L].actFun
if ! isequal(actFun, :softmax) && !(actFun==:σ &&
lossFun==:binaryCrossentropy)
dA[L] = eval(:($dlossFun.($A[$L], $Y))) #.* eval(:($dActFun.(Z[L])))
end
if layers[L].keepProb < 1.0 #to save time of multiplication in case keepProb was one
dA[L] = dA[L] .* D[L]
dA[L] = dA[L] ./ layers[L].keepProb
end
for l=L:-1:2
actFun = layers[l].actFun
dActFun = Symbol("d",actFun)
if l==L && (isequal(actFun, :softmax) ||
(actFun==:σ && lossFun==:binaryCrossentropy))
dZ[l] = A[l] .- Y
else
dZ[l] = dA[l] .* eval(:($dActFun.($Z[$l])))
end
dW[l] = dZ[l]*A[l-1]' ./m
if regulization > 0
if regulization==1
dW[l] .+= (λ/2m)
else
dW[l] .+= (λ/m) .* W[l]
end
end
dB[l] = 1/m .* sum(dZ[l], dims=2)
dA[l-1] = W[l]'dZ[l]
if layers[l-1].keepProb < 1.0 #to save time of multiplication in case keepProb was one
dA[l-1] = dA[l-1] .* D[l-1]
dA[l-1] = dA[l-1] ./ layers[l-1].keepProb
end
end
l=1 #shortcut cause I just copied from the for loop
actFun = layers[l].actFun
dActFun = Symbol("d",actFun)
dZ[l] = dA[l] .* eval(:($dActFun.($Z[$l])))
dW[l] = 1/m .* dZ[l]*X' #where X = A[0]
if regulization > 0
if regulization==1
dW[l] .+= (λ/2m)
else
dW[l] .+= (λ/m) .* W[l]
end
end
dB[l] = 1/m .* sum(dZ[l], dims=2)
grads = Dict("dW"=>dW,
"dB"=>dB)
return grads
end #backProp
function updateParams(model::Model, grads::Dict, tMiniBatch::Integer)
W, B, V, S, layers = model.W, model.B, model.V, model.S, model.layers
optimizer = model.optimizer
dW, dB = grads["dW"], grads["dB"]
L = length(layers)
α = model.α
β1, β2, ϵAdam = model.β1, model.β2, model.ϵAdam
#initialize the needed variables to hold the corrected values
#it is being init here cause these are not needed elsewhere
VCorrected, SCorrected = deepInitVS(W,B,optimizer)
if optimizer==:adam || optimizer==:momentum
for i=1:L
V[:dw][i] .= β1 .* V[:dw][i] .+ (1-β1) .* dW[i]
V[:db][i] .= β1 .* V[:db][i] .+ (1-β1) .* dB[i]
##correcting
VCorrected[:dw][i] .= V[:dw][i] ./ (1-β1^tMiniBatch)
VCorrected[:db][i] .= V[:db][i] ./ (1-β1^tMiniBatch)
if optimizer==:adam
S[:dw][i] .= β2 .* S[:dw][i] .+ (1-β2) .* (dW[i].^2)
S[:db][i] .= β2 .* S[:db][i] .+ (1-β2) .* (dB[i].^2)
##correcting
SCorrected[:dw][i] .= S[:dw][i] ./ (1-β2^tMiniBatch)
SCorrected[:db][i] .= S[:db][i] ./ (1-β2^tMiniBatch)
##update parameters with adam
W[i] .-= (α .* (VCorrected[:dw][i] ./ (sqrt.(SCorrected[:dw][i]) .+ ϵAdam)))
B[i] .-= (α .* (VCorrected[:db][i] ./ (sqrt.(SCorrected[:db][i]) .+ ϵAdam)))
else#if optimizer==:momentum
W[i] .-= (α .* VCorrected[:dw][i])
B[i] .-= (α .* VCorrected[:db][i])
end #if optimizer==:adam
end #for i=1:L
else
W .-= (α .* dW)
B .-= (α .* dB)
end #if optimizer==:adam || optimizer==:momentum
return W, B
end #updateParams
function updateParams!(model::Model, grads::Dict, tMiniBatch::Integer)
W, B, V, S, layers = model.W, model.B, model.V, model.S, model.layers
optimizer = model.optimizer
dW, dB = grads["dW"], grads["dB"]
L = length(layers)
α = model.α
β1, β2, ϵAdam = model.β1, model.β2, model.ϵAdam
#initialize the needed variables to hold the corrected values
#it is being init here cause these are not needed elsewhere
VCorrected, SCorrected = deepInitVS(W,B,optimizer)
if optimizer==:adam || optimizer==:momentum
for i=1:L
V[:dw][i] .= β1 .* V[:dw][i] .+ (1-β1) .* dW[i]
V[:db][i] .= β1 .* V[:db][i] .+ (1-β1) .* dB[i]
##correcting
VCorrected[:dw][i] .= V[:dw][i] ./ (1-β1^tMiniBatch)
VCorrected[:db][i] .= V[:db][i] ./ (1-β1^tMiniBatch)
if optimizer==:adam
S[:dw][i] .= β2 .* S[:dw][i] .+ (1-β2) .* (dW[i].^2)
S[:db][i] .= β2 .* S[:db][i] .+ (1-β2) .* (dB[i].^2)
##correcting
SCorrected[:dw][i] .= S[:dw][i] ./ (1-β2^tMiniBatch)
SCorrected[:db][i] .= S[:db][i] ./ (1-β2^tMiniBatch)
##update parameters with adam
W[i] .-= (α .* (VCorrected[:dw][i] ./ (sqrt.(SCorrected[:dw][i]) .+ ϵAdam)))
B[i] .-= (α .* (VCorrected[:db][i] ./ (sqrt.(SCorrected[:db][i]) .+ ϵAdam)))
else#if optimizer==:momentum
W[i] .-= (α .* VCorrected[:dw][i])
B[i] .-= (α .* VCorrected[:db][i])
end #if optimizer==:adam
end #for i=1:L
else
W .-= (α .* dW)
B .-= (α .* dB)
end #if optimizer==:adam || optimizer==:momentum
model.W, model.B = W, B
return
end #updateParams!
function updateParams!(model::Model,
cLayer::Layer,
cnt::Integer = -1;
tMiniBatch::Integer = 1)
optimizer = model.optimizer
α = model.α
β1, β2, ϵAdam = model.β1, model.β2, model.ϵAdam
if cLayer.updateCount >= cnt
return nothing
end #if cLayer.updateCount >= cnt
cLayer.updateCount += 1
#initialize the needed variables to hold the corrected values
#it is being init here cause these are not needed elsewhere
VCorrected = Dict(:dw=>similar(cLayer.dW), :db=>similar(cLayer.dB))
SCorrected = Dict(:dw=>similar(cLayer.dW), :db=>similar(cLayer.dB))
if optimizer==:adam || optimizer==:momentum
cLayer.V[:dw] .= β1 .* cLayer.V[:dw] .+ (1-β1) .* cLayer.dW
cLayer.V[:db] .= β1 .* cLayer.V[:db] .+ (1-β1) .* cLayer.dB
##correcting
VCorrected[:dw] .= cLayer.V[:dw] ./ (1-β1^tMiniBatch)
VCorrected[:db] .= cLayer.V[:db] ./ (1-β1^tMiniBatch)
if optimizer==:adam
cLayer.S[:dw] .= β2 .* cLayer.S[:dw] .+ (1-β2) .* (cLayer.dW.^2)
cLayer.S[:db] .= β2 .* cLayer.S[:db] .+ (1-β2) .* (cLayer.dB.^2)
##correcting
SCorrected[:dw] .= cLayer.S[:dw] ./ (1-β2^tMiniBatch)
SCorrected[:db] .= cLayer.S[:db] ./ (1-β2^tMiniBatch)
##update parameters with adam
cLayer.W .-= (α .* (VCorrected[:dw] ./ (sqrt.(SCorrected[:dw]) .+ ϵAdam)))
cLayer.B .-= (α .* (VCorrected[:db] ./ (sqrt.(SCorrected[:db]) .+ ϵAdam)))
else#if optimizer==:momentum
cLayer.W .-= (α .* VCorrected[:dw])
cLayer.B .-= (α .* VCorrected[:db])
end #if optimizer==:adam
else
cLayer.W .-= (α .* cLayer.dW)
cLayer.B .-= (α .* cLayer.dB)
end #if optimizer==:adam || optimizer==:momentum
return nothing
end #updateParams!
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 315 | """
This file holds all the includes commands for easier test and dev purpose
"""
include("TypeDef.jl")
include("lossFuns.jl")
include("actFuns.jl")
include("initializations.jl")
include("cnn/includes.jl")
# include("backForProp.jl")
include("parallelBackForProp.jl")
include("assistance.jl")
include("chain.jl")
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 6578 |
### single layer initWB
#TODO create initializers dependent for each method
"""
initialize W and B for layer with inputs of size of (nl_1) and layer size
of (nl)
returns:
W: of size of (nl, nl_1)
"""
function initWB!(
cLayer::FCLayer,
p::Type{T} = Float64::Type{Float64};
He = true,
coef = 0.01,
zro = false,
) where {T}
s = (cLayer.channels, cLayer.prevLayer.channels)
if He
coef = sqrt(2 / s[end])
end
if zro
W = zeros(T, s...)
else
W = T.(randn(s...) .* coef)
end
B = zeros(T, (s[1], 1))
cLayer.W, cLayer.B = W, B
cLayer.dW = zeros(T, s...)
cLayer.dB = deepcopy(B)
return nothing
end #initWB
function initWB!(
cLayer::Activation,
p::Type{T} = Float64::Type{Float64};
He = true,
coef = 0.01,
zro = false,
) where {T}
return nothing
end
function initWB!(
cLayer::Flatten,
p::Type{T} = Float64::Type{Float64};
He = true,
coef = 0.01,
zro = false,
) where {T}
return nothing
end
function initWB!(
cLayer::ConcatLayer,
p::Type{T} = Float64::Type{Float64};
He = true,
coef = 0.01,
zro = false,
) where {T}
return nothing
end
function initWB!(
cLayer::Input,
p::Type{T} = Float64::Type{Float64};
He = true,
coef = 0.01,
zro = false,
) where {T}
# cLayer.inputS = cLayer.outputS = size(cLayer.A)
return nothing
end
function initWB!(
cLayer::BatchNorm,
p::Type{T} = Float64::Type{Float64};
He = true,
coef = 0.01,
zro = false,
) where {T}
cn = cLayer.prevLayer.channels
if He
coef = sqrt(2 / cn)
end
N = length(cLayer.prevLayer.inputS)
normDim = cLayer.dim
#bring the batch size into front
S = (cLayer.prevLayer.outputS[end], cLayer.prevLayer.outputS[1:end-1]...)
paramS = Array{Integer,1}([1])
for i=2:N
if S[i] < 1 || (i-1) <= normDim
push!(paramS, 1)
else
push!(paramS, S[i])
end #if S[i] < 1
end #for
if !zro
W = T.(randn(paramS...) .* coef)
else
W = zeros(T, paramS...)
end
if !(size(cLayer.W) == size(W))
cLayer.W = W
cLayer.dW = zeros(T, paramS...)
cLayer.B = zeros(T, paramS...)
cLayer.dB = zeros(T, paramS...)
end #if !(size(cLayer.W) == size(W))
return nothing
end #function initWB!(cLayer::BatchNorm
export initWB!
### deepInitWB!
"""
initialize W's and B's using
inputs:
X := is the input of the neural Network
outLayer := is the output Layer or the current layer
of initialization
cnt := is a counter to determinde the current step
and its an internal variable
kwargs:
He := is a true/false array, whether to use the He **et al.** initialization
or not
coef := when not using He **et al.** initialization use this coef
to multiply with the random numbers initialization
zro := true/false variable whether to initialize W with zeros or not
"""
function deepInitWB!(
outLayer::Layer,
cnt = -1;
He = true,
coef = 0.01,
zro = false,
dtype = Float64,
)
if cnt < 0
cnt = outLayer.forwCount + 1
end
if dtype == nothing
T = eltype(X)
else
T = dtype
end
prevLayer = outLayer.prevLayer
forwCount = outLayer.forwCount
if outLayer isa Input
if forwCount < cnt
outLayer.forwCount += 1
initWB!(outLayer, T; He = He, coef = coef, zro = zro)
end #if forwCount < cnt
elseif isa(outLayer, MILayer)
if forwCount < cnt
outLayer.forwCount += 1
for prevLayer in outLayer.prevLayer
deepInitWB!(
prevLayer,
cnt;
He = He,
coef = coef,
zro = zro,
dtype = dtype,
)
end #for prevLayer in outLayer.prevLayer
end #if forwCount < cnt
else #if prevLayer == nothing
if forwCount < cnt
outLayer.forwCount += 1
deepInitWB!(
prevLayer,
cnt;
He = He,
coef = coef,
zro = zro,
dtype = dtype,
)
initWB!(outLayer, T; He = He, coef = coef, zro = zro)
end #if forwCount < cnt
end #if prevLayer == nothing
return nothing
end #deepInitWB
export deepInitWB!
### single layer initVS
function initVS!(
cLayer::FCLayer,
optimizer::Symbol
)
cLayer.V[:dw] = deepcopy(cLayer.dW)
cLayer.V[:db] = deepcopy(cLayer.dB)
if optimizer == :adam
cLayer.S = deepcopy(cLayer.V)
end #if optimizer == :adam
return nothing
end #function initVS!
function initVS!(
cLayer::IoA,
optimizer::Symbol
) where {IoA <: Union{Input, Activation, Flatten, ConcatLayer}}
return nothing
end #
function initVS!(
cLayer::BatchNorm,
optimizer::Symbol,
)
T = typeof(cLayer.W)
if (! haskey(cLayer.V, :dw)) || (size(cLayer.V[:dw]) != size(cLayer.dW))
cLayer.V = Dict(:dw=>deepcopy(cLayer.dW), :db=>deepcopy(cLayer.dB))
cLayer.S = deepcopy(cLayer.V)
end
return nothing
end
export initVS!
### deepInitVS!
function deepInitVS!(outLayer::Layer, optimizer::Symbol, cnt::Integer = -1)
if cnt < 0
cnt = outLayer.forwCount + 1
end
prevLayer = outLayer.prevLayer
if optimizer == :adam || optimizer == :momentum
if outLayer isa Input
if outLayer.forwCount < cnt
outLayer.forwCount += 1
initVS!(outLayer, optimizer)
end #if outLayer.forwCount < cnt
elseif isa(outLayer, MILayer)
if outLayer.forwCount < cnt
outLayer.forwCount += 1
for prevLayer in outLayer.prevLayer
deepInitVS!(prevLayer, optimizer, cnt)
end #for
end #if outLayer.forwCount < cnt
else #if prevLayer == nothing
if outLayer.forwCount < cnt
outLayer.forwCount += 1
deepInitVS!(prevLayer, optimizer, cnt)
initVS!(outLayer, optimizer)
end #if outLayer.forwCount < cnt
end #if prevLayer == nothing
end #if optimizer==:adam || optimizer==:momentum
return nothing
end #function deepInitVS!
export deepInitVS!
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 8557 |
"""
Depricated file
"""
function layerBackProp(
cLayer::FCLayer,
model::Model,
actFun::SoS,
labels::AbstractArray,
) where {SoS<:Union{Type{softmax},Type{σ}}}
lossFun = model.lossFun
dlossFun = Symbol("d", lossFun)
A = cLayer.A
Y = labels
dZ = eval(:($dlossFun($A, $Y)))
cLayer.dA = cLayer.W'dZ
return dZ
end #softmax or σ layerBackProp
function layerBackProp(
cLayer::Activation,
model::Model,
actFun::SoS,
labels::AbstractArray,
) where {SoS<:Union{Type{softmax},Type{σ}}}
lossFun = model.lossFun
dlossFun = Symbol("d", lossFun)
A = cLayer.A
Y = labels
dZ = eval(:($dlossFun($A, $Y)))
return dZ
end #softmax or σ layerBackProp
function layerBackProp!(
cLayer::FCLayer,
model::Model,
dA::AoN = nothing;
labels::AoN = nothing,
kwargs...,
) where {AoN<:Union{AbstractArray,Nothing}}
prevLayer = cLayer.prevLayer
lossFun = model.lossFun
m = size(cLayer.A)[end]
A = cLayer.A
regulization, λ = model.regulization, model.λ
actFun = cLayer.actFun
dZ = []
if cLayer.actFun == model.outLayer.actFun
dZ = layerBackProp(cLayer, model, eval(:($actFun)), labels)
elseif dA != nothing
keepProb = cLayer.keepProb
if keepProb < 1.0 #to save time of multiplication in case keepProb was one
D = rand(cLayer.channels, 1) .< keepProb
dA .*= D
dA ./= keepProb
end
dActFun = Symbol("d", cLayer.actFun)
Z = cLayer.Z
dZ = dA .* eval(:($dActFun($Z)))
elseif all(
i -> (i.backCount == cLayer.nextLayers[1].backCount),
cLayer.nextLayers,
)
dA = []
for nextLayer in cLayer.nextLayers
try
dA .+= nextLayer.dA
catch e #in case first time DimensionMismatch
dA = nextLayer.dA
end
end #for
keepProb = cLayer.keepProb
if keepProb < 1.0 #to save time of multiplication in case keepProb was one
D = rand(cLayer.channels, 1) .< keepProb
dA .*= D
dA ./= keepProb
end
dActFun = Symbol("d", cLayer.actFun)
Z = cLayer.Z
dZ = dA .* eval(:($dActFun($Z)))
else #in case not every next layer done backprop
return nothing
end #if all(i->(i.backCount==cLayer.nextLayers[1].backCount), cLayer.nextLayers)
cLayer.dW = dZ * cLayer.prevLayer.A' ./ m
if regulization > 0
if regulization == 1
cLayer.dW .+= (λ / 2m)
else
cLayer.dW .+= (λ / m) .* cLayer.W
end
end
cLayer.dB = 1 / m .* sum(dZ, dims = 2)
cLayer.dA = cLayer.W'dZ
cLayer.backCount += 1
return nothing
end #function layerBackProp(cLayer::FCLayer)
function layerBackProp!(
cLayer::Activation,
model::Model,
dA::AoN = nothing;
labels::AoN = nothing,
kwargs...,
) where {AoN<:Union{AbstractArray,Nothing}}
prevLayer = cLayer.prevLayer
lossFun = model.lossFun
m = size(cLayer.A)[end]
regulization, λ = model.regulization, model.λ
actFun = cLayer.actFun
dZ = []
if cLayer.actFun == model.outLayer.actFun
dZ = layerBackProp(cLayer, model, eval(:($actFun)), labels)
elseif dA != nothing
keepProb = cLayer.keepProb
if keepProb < 1.0 #to save time of multiplication in case keepProb was one
D = rand(cLayer.channels, 1) .< keepProb
dA .*= D
dA ./= keepProb
end
dActFun = Symbol("d", cLayer.actFun)
Z = cLayer.Z
dZ = dA .* eval(:($dActFun($Z)))
elseif all(
i -> (i.backCount == cLayer.nextLayers[1].backCount),
cLayer.nextLayers,
)
dA = []
for nextLayer in cLayer.nextLayers
try
dA .+= nextLayer.dA
catch e #if first time DimensionMismatch
dA = nextLayer.dA
end #try/catch
end #for
try
keepProb = cLayer.keepProb
if keepProb < 1.0 #to save time of multiplication in case keepProb was one
D = rand(cLayer.channels, 1) .< keepProb
dA .*= D
dA ./= keepProb
end
catch e
end
dActFun = Symbol("d", cLayer.actFun)
Z = cLayer.prevLayer.A #this is the Activation layer
dZ = dA .* eval(:($dActFun($Z)))
else #in case not every next layer done backprop
return nothing
end #if all(i->(i.backCount==cLayer.nextLayers[1].backCount), cLayer.nextLayers)
cLayer.dA = dZ
cLayer.backCount += 1
return nothing
end #function layerBackProp!(cLayer::Activation
function layerBackProp!(
cLayer::AddLayer,
model::Model,
dA::AoN = nothing;
labels::AoN = nothing,
kwargs...,
) where {AoN<:Union{AbstractArray,Nothing}}
# m = size(cLayer.A)[end]
#
# A = cLayer.A
#
# regulization, λ = model.regulization, model.λ
if dA != nothing
keepProb = cLayer.keepProb
if keepProb < 1.0 #to save time of multiplication in case keepProb was one
D = rand(cLayer.channels, 1) .< keepProb
dA .*= D
dA ./= keepProb
end
dActFun = Symbol("d", cLayer.actFun)
Z = cLayer.Z
dZ = dA .* eval(:($dActFun($Z)))
elseif all(
i -> (i.backCount == cLayer.nextLayers[1].backCount),
cLayer.nextLayers,
)
dA = []
for nextLayer in cLayer.nextLayers
try
dA .+= nextLayer.dA
catch e
dA = nextLayer.dA #to initialize dA
end #try/catch
end #for
cLayer.dA = dA
else #in case not every next layer done backprop
return nothing
end #if all(i->(i.backCount==cLayer.nextLayers[1].backCount), cLayer.nextLayers)
cLayer.backCount += 1
return nothing
end #function layerBackProp!(cLayer::AddLayer
function layerBackProp!(
cLayer::Input,
model::Model,
dA::AoN = nothing;
labels::AoN = nothing,
kwargs...,
) where {AoN<:Union{AbstractArray,Nothing}}
# m = size(cLayer.A)[end]
#
# A = cLayer.A
#
# regulization, λ = model.regulization, model.λ
if dA != nothing
cLayer.dA = dA
elseif all(
i -> (i.backCount == cLayer.nextLayers[1].backCount),
cLayer.nextLayers,
)
dA = []
for nextLayer in cLayer.nextLayers
try
dA .+= nextLayer.dA
catch e
dA = nextLayer.dA #need to initialize dA
end #try/catch
end #for
cLayer.dA = dA
else #in case not every next layer done backprop
return nothing
end #if all(i->(i.backCount==cLayer.nextLayers[1].backCount), cLayer.nextLayers)
cLayer.backCount += 1
return nothing
end #function layerBackProp!(cLayer::Input
function layerBackProp!(
cLayer::BatchNorm,
model::Model,
dA::AoN = nothing;
labels::AoN = nothing,
kwargs...,
) where {AoN<:Union{AbstractArray,Nothing}}
prevLayer = cLayer.prevLayer
lossFun = model.lossFun
m = size(cLayer.A)[end]
A = cLayer.A
normDim = cLayer.dim
regulization, λ = model.regulization, model.λ
dZ = []
if dA != nothing
dZ = cLayer.W .* dA
elseif all(
i -> (i.backCount == cLayer.nextLayers[1].backCount),
cLayer.nextLayers,
)
dA = []
for nextLayer in cLayer.nextLayers
try
dA .+= nextLayer.dA
catch e
dA = nextLayer.dA #need to initialize dA
end #try/catch
end #for
Z = cLayer.Z
dZ = cLayer.W .* dA
else #in case not every next layer done backprop
return nothing
end #if all(i->(i.backCount==cLayer.nextLayers[1].backCount), cLayer.nextLayers)
cLayer.dW = sum(dZ .* cLayer.Z, dims = 1:normDim)
if regulization > 0
if regulization == 1
cLayer.dW .+= (λ / 2m)
else
cLayer.dW .+= (λ / m) .* cLayer.W
end
end
cLayer.dB = sum(dZ, dims = 1:normDim)
N = prod(size(dZ)[1:normDim])
varep = cLayer.var .+ cLayer.ϵ
dẐ =
dZ ./ sqrt.(varep) .*
(.-(cLayer.Ai_μ_s) .* ((N - 1) / N^2) ./ (varep) .^ 2 .+ 1) .*
(1 - 1 / N)
cLayer.dA = dẐ
cLayer.backCount += 1
return nothing
end #function layerBackProp(cLayer::BatchNorm)
export layerBackProp!
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 2313 |
"""
Depricated file
"""
using Statistics
###Input Layer
function layerForProp!(
cLayer::Input,
X::AbstractArray = Array{Any,1}(undef,0);
kwargs...,
)
if length(X) != 0
cLayer.A = X
cLayer.inputS = cLayer.outputS = size(X)
end
cLayer.forwCount += 1
# Base.GC.gc()
return nothing
end
###FCLayer forprop
function layerForProp!(
cLayer::FCLayer,
Ai::AbstractArray = Array{Any,1}(undef,0);
kwargs...,
)
if length(Ai) == 0
Ai = cLayer.prevLayer.A
end
cLayer.inputS = cLayer.prevLayer.outputS
cLayer.Z = cLayer.W * Ai .+ cLayer.B
actFun = cLayer.actFun
Z = cLayer.Z
cLayer.outputS = size(Z)
cLayer.A = eval(:($actFun($Z)))
cLayer.forwCount += 1
# Base.GC.gc()
return nothing
end #function layerForProp!(cLayer::FCLayer)
###AddLayer forprop
function layerForProp!(cLayer::AddLayer; kwargs...,)
cLayer.A = similar(cLayer.prevLayer[1].A)
cLayer.A .= 0
for prevLayer in cLayer.prevLayer
cLayer.A .+= prevLayer.A
end
cLayer.forwCount += 1
# Base.GC.gc()
return nothing
end #function layerForProp!(cLayer::AddLayer)
###Activation forprop
function layerForProp!(
cLayer::Activation,
Ai::AbstractArray = Array{Any,1}(undef,0);
kwargs...,
)
if length(Ai) == 0
Ai = cLayer.prevLayer.A
end
actFun = cLayer.actFun
cLayer.A = eval(:($actFun($Ai)))
cLayer.inputS = cLayer.outputS = size(Ai)
cLayer.forwCount += 1
# Ai = nothing
# Base.GC.gc()
return nothing
end #function layerForProp!(cLayer::Activation)
###BatchNorm
function layerForProp!(
cLayer::BatchNorm,
Ai::AbstractArray = Array{Any,1}(undef,0);
kwargs...,
)
if length(Ai) == 0
Ai = cLayer.prevLayer.A
end
initWB!(cLayer)
cLayer.μ = mean(Ai, dims = 1:cLayer.dim)
Ai_μ = Ai .- cLayer.μ
N = prod(size(Ai)[1:cLayer.dim])
cLayer.Ai_μ_s = Ai_μ .^ 2
cLayer.var = sum(cLayer.Ai_μ_s, dims = 1:cLayer.dim) ./ N
cLayer.Z = Ai_μ ./ sqrt.(cLayer.var .+ cLayer.ϵ)
cLayer.A = cLayer.W .* cLayer.Z .+ cLayer.B
cLayer.forwCount += 1
Ai_μ = nothing
cLayer.inputS = cLayer.outputS = size(Ai)
# Base.GC.gc()
return nothing
end #function layerForProp!(cLayer::BatchNorm)
export layerForProp!
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 3265 |
@doc raw"""
function layerUpdateParams!(
model::Model,
cLayer::FoB,
cnt::Integer = -1;
tMiniBatch::Integer = 1,
kwargs...,
) where {FoB <: Union{FCLayer, BatchNorm}}
update trainable parameters for `FCLayer` and `BatchNorm` layers
"""
function layerUpdateParams!(
model::Model,
cLayer::FoB,
cnt::Integer = -1;
tMiniBatch::Integer = 1,
kwargs...,
) where {FoB <: Union{FCLayer, BatchNorm}}
optimizer = model.optimizer
α = model.α
β1, β2, ϵAdam = model.β1, model.β2, model.ϵAdam
if cLayer.updateCount >= cnt
return nothing
end #if cLayer.updateCount >= cnt
if !all(i->(i.backCount==cLayer.nextLayers[1].backCount), cLayer.nextLayers)
return nothing
end
#initialize the needed variables to hold the corrected values
#it is being init here cause these are not needed elsewhere
VCorrected = Dict(:dw=>similar(cLayer.dW), :db=>similar(cLayer.dB))
SCorrected = Dict(:dw=>similar(cLayer.dW), :db=>similar(cLayer.dB))
if optimizer==:adam || optimizer==:momentum
if tMiniBatch == 1
cLayer.V[:dw] .= 0
cLayer.V[:db] .= 0
end
cLayer.V[:dw] .= β1 .* cLayer.V[:dw] .+ (1-β1) .* cLayer.dW
cLayer.V[:db] .= β1 .* cLayer.V[:db] .+ (1-β1) .* cLayer.dB
##correcting
VCorrected[:dw] .= cLayer.V[:dw] ./ (1-β1^tMiniBatch)
VCorrected[:db] .= cLayer.V[:db] ./ (1-β1^tMiniBatch)
if optimizer==:adam
if tMiniBatch == 1
cLayer.S[:dw] .= 0
cLayer.S[:db] .= 0
end
cLayer.S[:dw] .= β2 .* cLayer.S[:dw] .+ (1-β2) .* (cLayer.dW.^2)
cLayer.S[:db] .= β2 .* cLayer.S[:db] .+ (1-β2) .* (cLayer.dB.^2)
##correcting
SCorrected[:dw] .= cLayer.S[:dw] ./ (1-β2^tMiniBatch)
SCorrected[:db] .= cLayer.S[:db] ./ (1-β2^tMiniBatch)
##update parameters with adam
cLayer.W .-= (α .* (VCorrected[:dw] ./ (sqrt.(SCorrected[:dw]) .+ ϵAdam)))
cLayer.B .-= (α .* (VCorrected[:db] ./ (sqrt.(SCorrected[:db]) .+ ϵAdam)))
else#if optimizer==:momentum
cLayer.W .-= (α .* VCorrected[:dw])
cLayer.B .-= (α .* VCorrected[:db])
end #if optimizer==:adam
else
cLayer.W .-= (α .* cLayer.dW)
cLayer.B .-= (α .* cLayer.dB)
end #if optimizer==:adam || optimizer==:momentum
cLayer.updateCount += 1
return nothing
end #updateParams!
function layerUpdateParams!(
model::Model,
cLayer::IoA,
cnt::Integer = -1;
tMiniBatch::Integer = 1,
kwargs...,
) where {IoA <: Union{Input, Activation, AddLayer, Flatten, ConcatLayer}}
# optimizer = model.optimizer
# α = model.α
# β1, β2, ϵAdam = model.β1, model.β2, model.ϵAdam
if cLayer.updateCount >= cnt
return nothing
end #if cLayer.updateCount >= cnt
if !all(i->(i.updateCount==cLayer.nextLayers[1].updateCount), cLayer.nextLayers)
return nothing
end
cLayer.updateCount += 1
return nothing
end #updateParams!
export layerUpdateParams!
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 2391 |
abstract type lossFun end
export lossFun
### binaryCrossentropy
"""
return the average cross entropy loss over vector of labels and predictions
input:
a := (?1, c,m) matrix of predicted values, where c is the number of classes
y := (?1, c,m) matrix of predicted values, where c is the number of classes
Note: in case the number of classes is one (1) it is okay to have
a scaler values for a and y
output:
J := scaler value of the cross entropy loss
"""
abstract type binaryCrossentropy <: lossFun end
function binaryCrossentropy(a, y)
aNew = prevnextfloat.(a)
J = .-(y .* log.(aNew) .+ (1 .- y) .* log.(1 .- aNew))
return J
end #binaryCrossentropy
export binaryCrossentropy
"""
compute the drivative of cross-entropy loss function to the input of the
layer dZ
"""
function dbinaryCrossentropy(a, y)
dJ = a .- y
return dJ
end #dbinaryCrossentropy
export dbinaryCrossentropy
### categoricalCrossentropy
abstract type categoricalCrossentropy <: lossFun end
function categoricalCrossentropy(a, y)
aNew = prevnextfloat.(a)
J = .-(y .* log.(aNew))
return J
end
function dcategoricalCrossentropy(a, y)
dJ = a .- y
return dJ
end
export categoricalCrossentropy, dcategoricalCrossentropy
"""
return previous float if x == 1 and nextfloat if x == 0
"""
prevnextfloat(x) = x==0 ? nextfloat(x) : x==1 ? prevfloat(x) : x
export prevnextfloat
### cost function
"""
function cost(
loss::Type{categoricalCrossentropy},
A::AbstractArray{T1,N},
Y::AbstractArray{T2,N},
) where {T1, T2, N}
Compute the cost for `categoricalCrossentropy` loss function
"""
function cost(
loss::Type{categoricalCrossentropy},
A::AbstractArray{T1,N},
Y::AbstractArray{T2,N},
) where {T1, T2, N}
c, m = size(A)[N-1:N]
costs = sum(loss(A,Y)) / m
return Float64.(costs)
end #function cost
"""
function cost(
loss::Type{binaryCrossentropy},
A::AbstractArray{T1,N},
Y::AbstractArray{T2,N},
) where {T1, T2, N}
Compute the cost for `binaryCrossentropy` loss function
"""
function cost(
loss::Type{binaryCrossentropy},
A::AbstractArray{T1,N},
Y::AbstractArray{T2,N},
) where {T1, T2, N}
c, m = size(A)[N-1:N]
costs = sum(loss(A,Y)) / (c*m)
return Float64.(costs)
end #function cost
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 23696 | include("parallelLayerForProp.jl")
using ProgressMeter
try
ProgressMeter.ijulia_behavior(:clear)
catch
end
using Random
using LinearAlgebra
###
@doc raw"""
function chainForProp(
X::AbstractArray{T,N},
cLayer::Layer,
cnt::Integer = -1;
FCache = Dict{Layer,Dict{Symbol,AbstractArray}}(),
kwargs...,
) where {T,N}
perform the chained forward propagation using recursive calls
# Arguments:
- `X::AbstractArray{T,N}` := input of the input layer
- `cLayer::Layer` := Input Layer
- `cnt::Integer` := an internal counter used to cache the layers was performed not to redo it again
# Returns
- `Cache::Dict{Layer, Dict{Symbol, Array}}` := the output each layer either A, Z or together As Dict of layer to dict of Symbols and Arrays for internal use, it set again the values of Z and A in each layer to be used later in back propagation and add one to the layer forwCount value when pass through it
"""
function chainForProp(
X::AbstractArray{T,N},
cLayer::Layer,
cnt::Integer = -1;
FCache = Dict{Layer,Dict{Symbol,AbstractArray}}(),
kwargs...,
) where {T,N}
if cnt < 0
cnt = cLayer.forwCount + 1
end
if length(cLayer.nextLayers) == 0
if cLayer.forwCount < cnt
FCache[cLayer] = layerForProp(cLayer; FCache = FCache, kwargs...)
end #if cLayer.forwCount < cnt
return FCache
elseif isa(cLayer, MILayer) #if typeof(cLayer)==AddLayer
if all(
i -> (i.forwCount == cLayer.prevLayer[1].forwCount),
cLayer.prevLayer,
)
FCache[cLayer] = layerForProp(cLayer; FCache = FCache, kwargs...)
for nextLayer in cLayer.nextLayers
FCache =
chainForProp(X, nextLayer, cnt; FCache = FCache, kwargs...)
end
end #if all
return FCache
else #if cLayer.prevLayer==nothing
if cLayer.forwCount < cnt
if cLayer isa Input
FCache[cLayer] =
layerForProp(cLayer, X; FCache = FCache, kwargs...)
else
FCache[cLayer] =
layerForProp(cLayer; FCache = FCache, kwargs...)
end
for nextLayer in cLayer.nextLayers
FCache =
chainForProp(X, nextLayer, cnt; FCache = FCache, kwargs...)
end
end #if cLayer.forwCount < cnt
return FCache
end #if cLayer.prevLayer!=nothing
return FCache
end #function chainForProp!
export chainForProp
@doc raw"""
predictBatch(model::Model, X::AbstractArray, Y = nothing; kwargs...)
predict Y using the model and the input X and the labels Y
# Inputs
- `model::Model` := the trained model
- `X::AbstractArray` := the input `Array`
- `Y` := the input labels to compare with (optional)
# Output
- a `Tuple` of
* `Ŷ` := the predicted values
* `Ŷ_bool` := the predicted labels
* `"accuracy"` := the accuracy of the predicted labels
"""
function predictBatch(model::Model, X::AbstractArray, Y = nothing; kwargs...)
kwargs = Dict{Symbol, Any}(kwargs...)
kwargs[:prediction] = getindex(kwargs, :prediction, default = true)
FCache = chainForProp(X, model.inLayer; kwargs...)
Ŷ = FCache[model.outLayer][:A]
T = eltype(Ŷ)
outLayer = model.outLayer
actFun = outLayer.actFun
# if isbool(Y)
return Ŷ, probToValue(eval(:($actFun)), Ŷ; labels = Y)...
end #predict
export predictBatch
###
@doc raw"""
predict(model::Model, X_In::AbstractArray, Y_In = nothing; kwargs...)
Run the prediction based on the trained `model`
# Arguments
- `model::Model` := the trained `Model` to predict on
- `X_In` := the input `Array`
- `Y_In` := labels (optional) to evaluate the model
## Key-word Arugmets
- `batchSize` := default `32`
- `useProgBar` := (`Bool`) where or not to shoe the prograss bar
# Return
- a `Dict` of:
* `:YhatValue` := Array of the output of the integer prediction values
* `:YhatProb` := Array of the output probabilities
* `:accuracy` := the accuracy of prediction in case `Y_In` is given
"""
function predict(model::Model, X_In::AbstractArray, Y_In = nothing; kwargs...)
kwargs = Dict{Symbol, Any}(kwargs...)
batchSize = getindex(kwargs, :batchSize; default = 32)
printAcc = getindex(kwargs, :printAcc; default = true)
useProgBar = getindex(kwargs, :useProgBar; default = true)
GCInt = getindex(kwargs, :GCInt, default = 5)
noBool = getindex(kwargs, :noBool, default = false)
kwargs[:prediction] = getindex(kwargs, :prediction, default = true)
outLayer, lossFun, α = model.outLayer, model.lossFun, model.α
Costs = []
nX = ndims(X_In)
T = eltype(X_In)
m = size(X_In)[end]
# c = size(Y_train)[end-1]
nB = m ÷ batchSize
axX = axes(X_In)[1:end-1]
Y = nothing
if Y_In != nothing
axY = axes(Y_In)[1:end-1]
end
if useProgBar
p = Progress((m % batchSize != 0 ? nB + 1 : nB), 0.1)
end
nY = length(model.outLayer.outputS)
Ŷ_out =
Array{AbstractArray{T,nY},1}(undef, nB + ((m % batchSize == 0) ? 0 : 1))
Ŷ_prob_out =
Array{AbstractArray{T,nY},1}(undef, nB + ((m % batchSize == 0) ? 0 : 1))
accuracy =
Array{AbstractFloat,1}(undef, nB + ((m % batchSize == 0) ? 0 : 1))
# Threads.@threads
@simd for j = 1:nB
downInd = (j - 1) * batchSize + 1
upInd = j * batchSize
X = view(X_In, axX..., downInd:upInd)
if Y_In != nothing
Y = view(Y_In, axY..., downInd:upInd)
end
Ŷ_prob_out[j], Ŷ_out[j], accuracy[j] =
predictBatch(model, X, Y; kwargs...)
if useProgBar
update!(p, j, showvalues = [("Instances $m", j * batchSize)])
end #if useProgBar
# X = Y = nothing
# Ŷ = nothing
# if j%GCInt == 0
# Base.GC.gc()
# end
end
if m % batchSize != 0
downInd = (nB) * batchSize + 1
X = view(X_In, axX..., downInd:m)
if Y_In != nothing
Y = view(Y_In, axY..., downInd:m)
end
Ŷ_prob_out[nB+1], Ŷ_out[nB+1], accuracy[nB+1] =
predictBatch(model, X, Y; kwargs...)
# X = Y = nothing
# Base.GC.gc()
if useProgBar
update!(p, nB + 1, showvalues = [("Instances $m", m)])
end
end
# accuracyM = nothing
# if !(isempty(accuracy))
accuracyM = mean(filter(x -> x != nothing, accuracy))
# end
if noBool
Ŷ_values = nothing
Ŷ_prob = nothing
else
# Ŷ_values = Array{T,nY}(undef, repeat([0], nY)...)
Ŷ_values = cat(Ŷ_out... ; dims = nY)
Ŷ_prob = cat(Ŷ_prob_out...; dims = nY)
end
return Dict(
:YhatValue => Ŷ_values,
:YhatProb => Ŷ_prob,
:accuracy => accuracyM,
)
end #function predict(
# model::Model,
# X_In::AbstractArray,
# Y_In=nothing;
# batchSize = 32,
# printAcc = true,
# useProgBar = false,
# )
export predict
### chainBackProp
include("parallelLayerBackProp.jl")
# include("layerUpdateParams.jl")
@doc raw"""
function chainBackProp(
X::AbstractArray{T1,N1},
Y::AbstractArray{T2,N2},
model::Model,
FCache::Dict{Layer,Dict{Symbol,AbstractArray}},
cLayer::L = nothing,
BCache::Dict{Layer,Dict{Symbol,AbstractArray}}=Dict{Layer,Dict{Symbol,AbstractArray}}(),
cnt = -1;
tMiniBatch::Integer = -1, #can be used to perform both back and update params
kwargs...,
) where {L<:Union{Layer,Nothing},T1,T2,N1,N2}
# Arguments
- `X` := train data
- `Y` := train labels
- `model` := is the model to perform the back propagation on
- `FCache` := the cached values of the forward propagation as `Dict{Layer, Dict{Symbol, AbstractArray}}`
- `cLayer` := is an internal variable to hold the current layer
- `BCache` := to hold the cache of the back propagtion (internal variable)
- `cnt` := is an internal variable to count the step of back propagation currently on to avoid re-do it
## Key-word Arguments
- `tMiniBatch` := to perform both the back prop and update trainable parameters in the same recursive call (if less than 1 update during back propagation is ditched)
- `kwargs` := other key-word arguments to be bassed to `layerBackProp` methods
# Return
- `BCache` := the cached values of the back propagation
"""
function chainBackProp(
X::AbstractArray{T1,N1},
Y::AbstractArray{T2,N2},
model::Model,
FCache::Dict{Layer,Dict{Symbol,AbstractArray}},
cLayer::L = nothing,
BCache::Dict{Layer,Dict{Symbol,AbstractArray}}=Dict{Layer,Dict{Symbol,AbstractArray}}(),
cnt = -1;
tMiniBatch::Integer = -1, #can be used to perform both back and update params
kwargs...,
) where {L<:Union{Layer,Nothing},T1,T2,N1,N2}
if cnt < 0
cnt = model.outLayer.backCount + 1
end
if cLayer == nothing
cLayer = model.outLayer
BCache[cLayer] =
layerBackProp(cLayer, model, FCache, BCache; labels = Y, kwargs...)
if tMiniBatch > 0
layerUpdateParams!(
model,
cLayer,
cnt;
tMiniBatch = tMiniBatch,
kwargs...,
)
end
BCache = chainBackProp(
X,
Y,
model,
FCache,
cLayer.prevLayer,
BCache,
cnt;
tMiniBatch = tMiniBatch,
kwargs...,
)
elseif cLayer isa MILayer
BCache[cLayer] = layerBackProp(cLayer, model, FCache, BCache; kwargs...)
if tMiniBatch > 0
layerUpdateParams!(
model,
cLayer,
cnt;
tMiniBatch = tMiniBatch,
kwargs...,
)
end
if cLayer.backCount >= cnt #in case layerBackProp did not do the back
#prop becasue the next layers are not all
#done yet
for prevLayer in cLayer.prevLayer
BCache = chainBackProp(
X,
Y,
model,
FCache,
prevLayer,
BCache,
cnt;
tMiniBatch = tMiniBatch,
kwargs...,
)
end #for
end
else #if cLayer==nothing
BCache[cLayer] = layerBackProp(cLayer, model, FCache, BCache; kwargs...)
if tMiniBatch > 0
layerUpdateParams!(
model,
cLayer,
cnt;
tMiniBatch = tMiniBatch,
kwargs...,
)
end
if cLayer.backCount >= cnt #in case layerBackProp did not do the back
#prop becasue the next layers are not all
#done yet
if !(cLayer isa Input)
BCache = chainBackProp(
X,
Y,
model,
FCache,
cLayer.prevLayer,
BCache,
cnt;
tMiniBatch = tMiniBatch,
kwargs...,
)
end #if cLayer.prevLayer == nothing
end
end #if cLayer==nothing
return BCache
end #backProp
export chainBackProp
###update parameters
include("layerUpdateParams.jl")
@doc raw"""
chainUpdateParams!(model::Model,
cLayer::L=nothing,
cnt = -1;
tMiniBatch::Integer = 1) where {L<:Union{Layer,Nothing}}
Update trainable parameters using recursive call
# Arguments
- `model` := the model holds the training and update process
- `cLayer` := internal variable for recursive call holds the current layer
- `cnt` := an internal variable to hold the count of update in each layer not to re-do it
## Key-word Arguments
- `tMiniBatch` := the number of mini-batch of the total train collection
# Return
- `nothing`
"""
function chainUpdateParams!(model::Model,
cLayer::L=nothing,
cnt = -1;
tMiniBatch::Integer = 1) where {L<:Union{Layer,Nothing}}
if cnt < 0
cnt = model.outLayer.updateCount + 1
end
if cLayer==nothing
layerUpdateParams!(model, model.outLayer, cnt, tMiniBatch=tMiniBatch)
chainUpdateParams!(model, model.outLayer.prevLayer, cnt, tMiniBatch=tMiniBatch)
elseif cLayer isa MILayer
#update the AddLayer updateCounter
if cLayer.updateCount < cnt
cLayer.updateCount += 1
end
for prevLayer in cLayer.prevLayer
chainUpdateParams!(model, prevLayer, cnt, tMiniBatch=tMiniBatch)
end #for
else #if cLayer==nothing
layerUpdateParams!(model, cLayer, cnt, tMiniBatch=tMiniBatch)
if !(cLayer isa Input)
chainUpdateParams!(model, cLayer.prevLayer, cnt, tMiniBatch=tMiniBatch)
end #if cLayer.prevLayer == nothing
end #if cLayer==nothing
return nothing
end #function chainUpdateParams!
export chainUpdateParams!
### train
@doc raw"""
train(
X_train,
Y_train,
model::Model,
epochs;
testData = nothing,
testLabels = nothing,
kwargs...,
)
Repeat the trainging (forward/backward propagation and update parameters)
# Argument
- `X_train` := the training data
- `Y_train` := the training labels
- `model` := the model to train
- `epochs` := the number of repetitions of the training phase
# Key-word Arguments
- `testData` := to evaluate the training process over test data too
- `testLabels` := to evaluate the training process over test data too
- `batchSize` := the size of training when mini batch training
` `useProgBar` := (true, false) value to use prograss bar
- `kwargs` := other key-word Arguments to pass for the lower functions in hierarchy
# Return
- A `Dict{Symbol, Vector}` of:
* `:trainAccuracies` := an `Array` of the accuracies of training data at each epoch
* `:trainCosts` := an `Array` of the costs of training data at each epoch
* In case `testDate` and `testLabels` are givens:
+ `:testAccuracies` := an `Array` of the accuracies of test data at each epoch
+ `:testCosts` := an `Array` of the costs of test data at each epoch
"""
function train(
X_train,
Y_train,
model::Model,
epochs;
testData = nothing,
testLabels = nothing,
kwargs...,
)
kwargs = Dict{Symbol, Any}(kwargs...)
batchSize = getindex(kwargs, :batchSize; default = 32)
printCostsInterval = getindex(kwargs, :printCostsInterval; default = 0)
useProgBar = getindex(kwargs, :useProgBar; default = true)
embedUpdate = getindex(kwargs, :embedUpdate; default = true)
metrics = getindex(kwargs, :metrics; default = [:accuracy, :cost])
Accuracy = :accuracy in metrics
Cost = :cost in metrics
test = testData != nothing && testLabels != nothing
inLayer, outLayer, lossFun, α = model.inLayer, model.outLayer, model.lossFun, model.α
outAct = outLayer.actFun
m = size(X_train)[end]
c = size(Y_train)[end-1]
nB = m ÷ batchSize
N = ndims(X_train)
axX = axes(X_train)[1:end-1]
axY = axes(Y_train)[1:end-1]
nMiniBatch = (nB + Integer(m % batchSize != 0))
if useProgBar
p = Progress(epochs*nMiniBatch, 0.1)
showValues = Dict{String, Real}("Epoch $(epochs)"=>0,
"Instances $(m)"=>0,
)
end
if Accuracy
showValues["Train Accuracy"] = 0.0
end
if Cost
showValues["Train Cost"] = 0.0
end
if test
testAcc = []
testCost = []
showValues["Test Accuracy"] = 0.0
showValues["Test Cost"] = 0.0
end
Accuracies = []
Costs = []
for i=1:epochs
shufInd = randperm(m)
minCosts = [] #the costs of all mini-batches
minAcc = []
for j=1:nB
downInd = (j-1)*batchSize+1
upInd = j * batchSize
batchInd = shufInd[downInd:upInd]
X = X_train[axX..., batchInd]
Y = Y_train[axY..., batchInd]
FCache = chainForProp(X, inLayer; kwargs...)
a = FCache[outLayer][:A]
if Accuracy
_, acc = probToValue(eval(:($outAct)), a; labels = Y)
push!(minAcc, acc)
if useProgBar
tmpAcc = mean(minAcc)
showValues["Train Accuracy"] = round(tmpAcc ; digits=4)
end
end
if Cost
minCost = cost(eval(:($lossFun)), a, Y)
push!(minCosts, minCost)
if useProgBar
tmpCost = mean(minCosts)
showValues["Train Cost"] = round(tmpCost; digits = 4)
end
end
if embedUpdate
BCache = chainBackProp(X,Y,
model,
FCache;
tMiniBatch = j,
kwargs...)
else
BCache = chainBackProp(X,Y,
model,
FCache;
kwargs...)
chainUpdateParams!(model; tMiniBatch = j)
end #if embedUpdate
if useProgBar
showValues["Epoch $(epochs)"] = i
showValues["Instances $(m)"] = j*batchSize
update!(p, (i-1)*nMiniBatch+j; showvalues=[("Epoch $(epochs)", pop!(showValues, "Epoch $(epochs)")), ("Instances $(m)", pop!(showValues, "Instances $(m)")), showValues...])
end
# if :accuracy in metrics && :cost in metrics
# update!(p, ((i-1)*(nB + ((m % batchSize == 0) ? 0 : 1)))+j; showvalues=[("Epoch ($epochs)", i), ("Instances ($m)", j*batchSize), (:Accuracy, round(mean(minAcc); digits=4)), (:Cost, round(mean(minCosts); digits=4))])
# elseif :accuracy in metrics
# update!(p, ((i-1)*(nB + ((m % batchSize == 0) ? 0 : 1)))+j; showvalues=[("Epoch ($epochs)", i), ("Instances ($m)", j*batchSize), (:Accuracy, round(mean(minAcc); digits=4))])
# elseif :cost in metrics
# update!(p, ((i-1)*(nB + ((m % batchSize == 0) ? 0 : 1)))+j; showvalues=[("Epoch ($epochs)", i), ("Instances ($m)", j*batchSize), (:Cost, round(mean(minCosts); digits=4))])
# else
# update!(p, ((i-1)*(nB + ((m % batchSize == 0) ? 0 : 1)))+j; showvalues=[("Epoch ($epochs)", i), ("Instances ($m)", j*batchSize)])
# end
# end
# chainUpdateParams!(model; tMiniBatch = j)
end #for j=1:nB iterate over the mini batches
if m%batchSize != 0
downInd = (nB)*batchSize+1
batchInd = shufInd[downInd:end]
X = X_train[axX..., batchInd]
Y = Y_train[axY..., batchInd]
FCache = chainForProp(X, inLayer; kwargs...)
if Accuracy
_, acc = probToValue(eval(:($outAct)), FCache[outLayer][:A]; labels = Y)
push!(minAcc, acc)
if useProgBar
tmpAcc = mean(minAcc)
showValues["Train Cost"] = round(tmpAcc ; digits=4)
end
end
a = FCache[outLayer][:A]
if Cost
minCost = cost(eval(:($lossFun)), a, Y)
push!(minCosts, minCost)
if useProgBar
tmpCost = mean(minCosts)
showValues["Train Cost"] = round(tmpCost; digits = 4)
end
end
if embedUpdate
BCache = chainBackProp(X,Y,
model,
FCache;
tMiniBatch = nB + 1,
kwargs...)
else
BCache = chainBackProp(X,Y,
model,
FCache;
kwargs...)
chainUpdateParams!(model; tMiniBatch = nB + 1)
end #if embedUpdate
end
if Accuracy
tmpAcc = mean(minAcc)
push!(Accuracies, tmpAcc)
if useProgBar
showValues["Train Accuracy"] = round(tmpAcc; digits=4)
end
end
if Cost
tmpCost = mean(minCosts)
push!(Costs, tmpCost)
if useProgBar
showValues["Train Cost"] = round(tmpCost; digits=4)
end
end
if printCostsInterval>0 && i%printCostsInterval==0
println("N = $i, Cost = $(Costs[end])")
end
if test
testDict = predict(model, testData, testLabels; useProgBar = false, batchSize=batchSize)
testcost = cost(eval(:($lossFun)), testDict[:YhatProb], testLabels)
if Accuracy
push!(testAcc, testDict[:accuracy])
if useProgBar
showValues["Test Accuracy"] = round(testDict[:accuracy]; digits=4)
end
end
if Cost
push!(testCost, testcost)
if useProgBar
showValues["Test Cost"] = round(testcost; digits=4)
end
end
end
if useProgBar
showValues["Epoch $(epochs)"] = i
showValues["Instances $(m)"] = m
update!(p, (i-1)*nMiniBatch+(nB+1); showvalues=[("Epoch $(epochs)", pop!(showValues, "Epoch $(epochs)")), ("Instances $(m)", pop!(showValues, "Instances $(m)")), showValues...])
end
# if useProgBar
# if useProgBar
# if :accuracy in metrics && :cost in metrics
# update!(p, ((i-1)*(nB + ((m % batchSize == 0) ? 0 : 1)))+(nB + 1); showvalues=[("Epoch ($epochs)", i), ("Instances ($m)", m), (:Accuracy, round(mean(minAcc); digits=4)), (:Cost, round(mean(minCosts); digits=4))])
# elseif :accuracy in metrics
# update!(p, ((i-1)*(nB + ((m % batchSize == 0) ? 0 : 1)))+(nB + 1); showvalues=[("Epoch ($epochs)", i), ("Instances ($m)", j*batchSize), (:Accuracy, round(mean(minAcc); digits=4))])
# elseif :cost in metrics
# update!(p, ((i-1)*(nB + ((m % batchSize == 0) ? 0 : 1)))+(nB + 1); showvalues=[("Epoch ($epochs)", i), ("Instances ($m)", j*batchSize), (:Cost, round(mean(minCosts); digits=4))])
# else
# update!(p, ((i-1)*(nB + ((m % batchSize == 0) ? 0 : 1)))+(nB + 1); showvalues=[("Epoch ($epochs)", i), ("Instances ($m)", j*batchSize)])
# end
# end
# end
end
# model.W, model.B = W, B
outDict = Dict{Symbol, Array{T,1} where {T}}()
if Accuracy
outDict[:trainAccuracies] = Accuracies
if test
outDict[:testAccuracies] = testAcc
end
end
if Cost
outDict[:trainCosts] = Costs
if test
outDict[:testCosts] = testCost
end
end
return outDict
end #train
export train
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 17716 |
@doc raw"""
layerBackProp(
cLayer::FCLayer,
model::Model,
actFun::SoS,
Ao::AbstractArray,
labels::AbstractArray,
) where {SoS<:Union{Type{softmax},Type{σ}}}
For output `FCLayer` layers with softmax and sigmoid activation functions
"""
function layerBackProp(
cLayer::FCLayer,
model::Model,
actFun::SoS,
Ao::AbstractArray,
labels::AbstractArray,
) where {SoS<:Union{Type{softmax},Type{σ}}}
lossFun = model.lossFun
dlossFun = Symbol("d", lossFun)
Y = labels
dZ = eval(:($dlossFun($Ao, $Y)))
# dAi = cLayer.W'dZ
return dZ #, dAi
end #softmax or σ layerBackProp
@doc raw"""
layerBackProp(
cLayer::Activation,
model::Model,
actFun::SoS,
Ao::AbstractArray,
labels::AbstractArray,
) where {SoS<:Union{Type{softmax},Type{σ}}}
For output `Activation` layers with softmax and sigmoid activation functions
"""
function layerBackProp(
cLayer::Activation,
model::Model,
actFun::SoS,
Ao::AbstractArray,
labels::AbstractArray,
) where {SoS<:Union{Type{softmax},Type{σ}}}
lossFun = model.lossFun
dlossFun = Symbol("d", lossFun)
Y = labels
dZ = eval(:($dlossFun($Ao, $Y)))
return dZ
end #softmax or σ layerBackProp
@doc raw"""
layerBackProp(
cLayer::FCLayer,
model::Model,
FCache::Dict{Layer, Dict{Symbol, AbstractArray}},
BCache::Dict{Layer, Dict{Symbol, AbstractArray}},
dAo::AbstractArray{T1,2} = Array{Any,2}(undef,0,0);
labels::AbstractArray{T2,2} = Array{Any,2}(undef,0,0),
kwargs...,
) where {T1,T2}
Perform the back propagation of `FCLayer` type on the activations and trainable parameters `W` and `B`
# Argument
- `cLayer` := the layer to perform the backprop on
- `model` := the `Model`
- `FCache` := the cache values of the forprop
- `BCache` := the cache values of the backprop from the front `Layer`(s)
- `dAo` := (for test purpose) the derivative of the front `Layer`
- `labels` := in case this is the output layer
# Return
- A `Dict{Symbol, AbstractArray}(:dA => dAi)`
"""
function layerBackProp(
cLayer::FCLayer,
model::Model,
FCache::Dict{Layer, Dict{Symbol, AbstractArray}},
BCache::Dict{Layer, Dict{Symbol, AbstractArray}},
dAo::AbstractArray{T1,2} = Array{Any,2}(undef,0,0);
labels::AbstractArray{T2,2} = Array{Any,2}(undef,0,0),
kwargs...,
) where {T1,T2}
prevLayer = cLayer.prevLayer
lossFun = model.lossFun
Ao = FCache[cLayer][:A]
regulization, λ = model.regulization, model.λ
actFun = cLayer.actFun
dZ = []
if cLayer.actFun == model.outLayer.actFun
dZ = layerBackProp(cLayer, model, eval(:($actFun)), Ao, labels)
elseif length(dAo) != 0
keepProb = cLayer.keepProb
if keepProb < 1.0 #to save time of multiplication in case keepProb was one
D = rand(cLayer.channels, 1) .< keepProb
dAo .*= D
dAo ./= keepProb
end
dActFun = Symbol("d", cLayer.actFun)
Z = FCache[cLayer][:Z]
dZ = dAo .* eval(:($dActFun($Z)))
elseif all(
i -> (i.backCount == cLayer.nextLayers[1].backCount),
cLayer.nextLayers,
)
dAo = []
for nextLayer in cLayer.nextLayers
try
dAo .+= getLayerSlice(cLayer, nextLayer, BCache)
catch e #in case first time DimensionMismatch
dAo = getLayerSlice(cLayer, nextLayer, BCache)
end
end #for
keepProb = cLayer.keepProb
if keepProb < 1.0 #to save time of multiplication in case keepProb was one
D = rand(cLayer.channels, 1) .< keepProb
dAo .*= D
dAo ./= keepProb
end
dActFun = Symbol("d", cLayer.actFun)
Z = FCache[cLayer][:Z]
dZ = dAo .* eval(:($dActFun($Z)))
else #in case not every next layer done backprop
return Dict(:dA => Array{Any,1}(undef,0))
end #if all(i->(i.backCount==cLayer.nextLayers[1].backCount), cLayer.nextLayers)
m = size(dZ)[end]
cLayer.dW = dZ * FCache[cLayer.prevLayer][:A]' ./ m
if regulization > 0
if regulization == 1
cLayer.dW .+= (λ / 2m)
else
cLayer.dW .+= (λ / m) .* cLayer.W
end
end
cLayer.dB = 1 / m .* sum(dZ, dims = 2)
dAi = cLayer.W'dZ
cLayer.backCount += 1
return Dict(:dA => dAi)
end #function layerBackProp(cLayer::FCLayer)
@doc raw"""
layerBackProp(
cLayer::Activation,
model::Model,
FCache::Dict{Layer, Dict{Symbol, AbstractArray}},
BCache::Dict{Layer, Dict{Symbol, AbstractArray}},
dAo::AbstractArray{T1,N} = Array{Any,1}(undef,0);
labels::AbstractArray{T2,N} = Array{Any,1}(undef,0),
kwargs...,
) where {T1,T2,N}
Perform the back propagation of `Activation` type
# Argument
- `cLayer` := the layer to perform the backprop on
- `model` := the `Model`
- `FCache` := the cache values of the forprop
- `BCache` := the cache values of the backprop from the front `Layer`(s)
- `dAo` := (for test purpose) the derivative of the front `Layer`
- `labels` := in case this is the output layer
# Return
- A `Dict{Symbol, AbstractArray}(:dA => dAi)`
"""
function layerBackProp(
cLayer::Activation,
model::Model,
FCache::Dict{Layer, Dict{Symbol, AbstractArray}},
BCache::Dict{Layer, Dict{Symbol, AbstractArray}},
dAo::AbstractArray{T1,N} = Array{Any,1}(undef,0);
labels::AbstractArray{T2,N} = Array{Any,1}(undef,0),
kwargs...,
) where {T1,T2,N}
prevLayer = cLayer.prevLayer
lossFun = model.lossFun
Ao = FCache[cLayer][:A]
regulization, λ = model.regulization, model.λ
actFun = cLayer.actFun
dZ = []
if cLayer.actFun == model.outLayer.actFun
dZ = layerBackProp(cLayer, model, eval(:($actFun)), Ao, labels)
elseif length(dAo) != 0
keepProb = cLayer.keepProb
if keepProb < 1.0 #to save time of multiplication in case keepProb was one
D = rand(cLayer.channels, 1) .< keepProb
dAo .*= D
dAo ./= keepProb
end
dActFun = Symbol("d", cLayer.actFun)
Z = FCache[cLayer][:Z]
dZ = dAo .* eval(:($dActFun($Z)))
elseif all(
i -> (i.backCount == cLayer.nextLayers[1].backCount),
cLayer.nextLayers,
)
dAo = []
for nextLayer in cLayer.nextLayers
try
dAo .+= getLayerSlice(cLayer, nextLayer, BCache)
catch e #in case first time DimensionMismatch
dAo = getLayerSlice(cLayer, nextLayer, BCache)
end
end #for
dActFun = Symbol("d", cLayer.actFun)
## get the input as the output of the previous layer
Z = FCache[cLayer.prevLayer][:A]
dZ = dAo .* eval(:($dActFun($Z)))
else #in case not every next layer done backprop
return Dict(:dA => Array{Any,1}(undef,0))
end #if all(i->(i.backCount==cLayer.nextLayers[1].backCount), cLayer.nextLayers)
# cLayer.dW = dZ * FCache[cLayer.prevLayer][:A]' ./ m
#
# if regulization > 0
# if regulization == 1
# cLayer.dW .+= (λ / 2m)
# else
# cLayer.dW .+= (λ / m) .* cLayer.W
# end
# end
#
# cLayer.dB = 1 / m .* sum(dZ, dims = 2)
dAi = dZ
cLayer.backCount += 1
return Dict(:dA => dAi)
end #function layerBackProp(cLayer::Activation
### Flatten
@doc raw"""
layerBackProp(
cLayer::Flatten,
model::Model,
FCache::Dict{Layer, Dict{Symbol, AbstractArray}},
BCache::Dict{Layer, Dict{Symbol, AbstractArray}},
dAo::AbstractArray{T1,N} = Array{Any,1}(undef,0);
labels::AbstractArray{T2,N} = Array{Any,1}(undef,0),
kwargs...,
) where {T1,T2,N}
Perform the back propagation of `Flatten` type
# Argument
- `cLayer` := the layer to perform the backprop on
- `model` := the `Model`
- `FCache` := the cache values of the forprop
- `BCache` := the cache values of the backprop from the front `Layer`(s)
- `dAo` := (for test purpose) the derivative of the front `Layer`
- `labels` := in case this is the output layer
# Return
- A `Dict{Symbol, AbstractArray}(:dA => dAi)`
"""
function layerBackProp(
cLayer::Flatten,
model::Model,
FCache::Dict{Layer, Dict{Symbol, AbstractArray}},
BCache::Dict{Layer, Dict{Symbol, AbstractArray}},
dAo::AbstractArray{T1,N} = Array{Any,1}(undef,0);
labels::AbstractArray{T2,N} = Array{Any,1}(undef,0),
kwargs...,
) where {T1,T2,N}
prevLayer = cLayer.prevLayer
Ao = FCache[cLayer][:A]
regulization, λ = model.regulization, model.λ
if length(dAo) == 0 && all(
i -> (i.backCount == cLayer.nextLayers[1].backCount),
cLayer.nextLayers,
)
dAo = []
for nextLayer in cLayer.nextLayers
try
dAo .+= getLayerSlice(cLayer, nextLayer, BCache)
catch e #in case first time DimensionMismatch
dAo = getLayerSlice(cLayer, nextLayer, BCache)
end
end #for
else #in case not every next layer done backprop
return Dict(:dA => Array{Any,1}(undef,0))
end #if all(i->(i.backCount==cLayer.nextLayers[1].backCount), cLayer.nextLayers)
dAi = reshape(dAo, cLayer.inputS)
cLayer.backCount += 1
return Dict(:dA => dAi)
end #function layerBackProp(cLayer::Activation
### AddLayer
@doc raw"""
layerBackProp(
cLayer::AddLayer,
model::Model,
FCache::Dict{Layer, Dict{Symbol, AbstractArray}},
BCache::Dict{Layer, Dict{Symbol, AbstractArray}},
dAo::AbstractArray{T1,N} = Array{Any,1}(undef,0);
labels::AbstractArray{T2,N} = Array{Any,1}(undef,0),
kwargs...,
) where {T1,T2,N}
Perform the back propagation of `AddLayer` type
# Argument
- `cLayer` := the layer to perform the backprop on
- `model` := the `Model`
- `FCache` := the cache values of the forprop
- `BCache` := the cache values of the backprop from the front `Layer`(s)
- `dAo` := (for test purpose) the derivative of the front `Layer`
- `labels` := in case this is the output layer
# Return
- A `Dict{Symbol, AbstractArray}(:dA => dAi)`
"""
function layerBackProp(
cLayer::AddLayer,
model::Model,
FCache::Dict{Layer, Dict{Symbol, AbstractArray}},
BCache::Dict{Layer, Dict{Symbol, AbstractArray}},
dAo::AbstractArray{T1,N} = Array{Any,1}(undef,0);
labels::AbstractArray{T2,N} = Array{Any,1}(undef,0),
kwargs...,
) where {T1,T2,N}
if length(dAo) != 0
return Dict(:dA => dAo)
elseif all(
i -> (i.backCount == cLayer.nextLayers[1].backCount),
cLayer.nextLayers,
)
dAo = []
for nextLayer in cLayer.nextLayers
try
dAo .+= getLayerSlice(cLayer, nextLayer, BCache)
catch e
dAo = getLayerSlice(cLayer, nextLayer, BCache) #to initialize dA
end #try/catch
end #for
else #in case not every next layer done backprop
return Dict(:dA => Array{Any,1}(undef,0))
end #if all(i->(i.backCount==cLayer.nextLayers[1].backCount), cLayer.nextLayers)
cLayer.backCount += 1
return Dict(:dA => dAo)
end #function layerBackProp(cLayer::AddLayer
### ConcatLayer
function layerBackProp(
cLayer::ConcatLayer,
model::Model,
FCache::Dict{Layer, Dict{Symbol, AbstractArray}},
BCache::Dict{Layer, Dict{Symbol, AbstractArray}},
dAo::AbstractArray{T1,N} = Array{Any,1}(undef,0);
labels::AbstractArray{T2,N} = Array{Any,1}(undef,0),
kwargs...,
) where {T1,T2,N}
if length(dAo) != 0
return Dict(:dA => dAo)
elseif all(
i -> (i.backCount == cLayer.nextLayers[1].backCount),
cLayer.nextLayers,
)
dAo = []
for nextLayer in cLayer.nextLayers
try
dAo .+= getLayerSlice(cLayer, nextLayer, BCache)
catch e
dAo = getLayerSlice(cLayer, nextLayer, BCache) #to initialize dA
end #try/catch
end #for
else #in case not every next layer done backprop
return Dict(:dA => Array{Any,1}(undef,0))
end #if all(i->(i.backCount==cLayer.nextLayers[1].backCount), cLayer.nextLayers)
cLayer.backCount += 1
return Dict(:dA => dAo)
end #function layerBackProp(cLayer::AddLayer
@doc raw"""
layerBackProp(
cLayer::Input,
model::Model,
FCache::Dict{Layer, Dict{Symbol, AbstractArray}},
BCache::Dict{Layer, Dict{Symbol, AbstractArray}},
dAo::AbstractArray{T1,N} = Array{Any,1}(undef,0);
labels::AbstractArray{T2,N} = Array{Any,1}(undef,0),
kwargs...,
) where {T1,T2,N}
Perform the back propagation of `Input` type
# Argument
- `cLayer` := the layer to perform the backprop on
- `model` := the `Model`
- `FCache` := the cache values of the forprop
- `BCache` := the cache values of the backprop from the front `Layer`(s)
- `dAo` := (for test purpose) the derivative of the front `Layer`
- `labels` := in case this is the output layer
# Return
- A `Dict{Symbol, AbstractArray}(:dA => dAi)`
"""
function layerBackProp(
cLayer::Input,
model::Model,
FCache::Dict{Layer, Dict{Symbol, AbstractArray}},
BCache::Dict{Layer, Dict{Symbol, AbstractArray}},
dAo::AbstractArray{T1,N} = Array{Any,1}(undef,0);
labels::AbstractArray{T2,N} = Array{Any,1}(undef,0),
kwargs...,
) where {T1,T2,N}
if length(dAo) != 0
return Dict(:dA => dAo)
elseif all(
i -> (i.backCount == cLayer.nextLayers[1].backCount),
cLayer.nextLayers,
)
dAo = []
for nextLayer in cLayer.nextLayers
try
dAo .+= getLayerSlice(cLayer, nextLayer, BCache)
catch e
dAo = getLayerSlice(cLayer, nextLayer, BCache) #need to initialize dA
end #try/catch
end #for
else #in case not every next layer done backprop
return Dict(:dA => Array{Any,1}(undef,0))
end #if all(i->(i.backCount==cLayer.nextLayers[1].backCount), cLayer.nextLayers)
cLayer.backCount += 1
return Dict(:dA => dAo)
end #function layerBackProp(cLayer::Input
@doc raw"""
layerBackProp(
cLayer::BatchNorm,
model::Model,
FCache::Dict{Layer, Dict{Symbol, AbstractArray}},
BCache::Dict{Layer, Dict{Symbol, AbstractArray}},
dAo::AbstractArray = Array{Any,1}(undef,0);
labels::AbstractArray = Array{Any,1}(undef,0),
kwargs...,
)
Perform the back propagation of `BatchNorm` type on the activations and trainable parameters `W` and `B`
# Argument
- `cLayer` := the layer to perform the backprop on
- `model` := the `Model`
- `FCache` := the cache values of the forprop
- `BCache` := the cache values of the backprop from the front `Layer`(s)
- `dAo` := (for test purpose) the derivative of the front `Layer`
- `labels` := in case this is the output layer
# Return
- A `Dict{Symbol, AbstractArray}(:dA => dAi)`
"""
function layerBackProp(
cLayer::BatchNorm,
model::Model,
FCache::Dict{Layer, Dict{Symbol, AbstractArray}},
BCache::Dict{Layer, Dict{Symbol, AbstractArray}},
dAo::AbstractArray = Array{Any,1}(undef,0);
labels::AbstractArray = Array{Any,1}(undef,0),
kwargs...,
)
prevLayer = cLayer.prevLayer
lossFun = model.lossFun
Ao = FCache[cLayer][:A]
Ai = FCache[cLayer.prevLayer][:A]
m = size(Ao)[end]
normDim = cLayer.dim+1
regulization, λ = model.regulization, model.λ
N = length(cLayer.outputS)
dZ = []
if length(dAo) != 0
dAo = permutedims(dAo, [N, (1:N-1)...])
dZ = cLayer.W .* dAo
elseif all(
i -> (i.backCount == cLayer.nextLayers[1].backCount),
cLayer.nextLayers,
)
dAo = []
for nextLayer in cLayer.nextLayers
try
dAo .+= getLayerSlice(cLayer, nextLayer, BCache)
catch e
dAo = getLayerSlice(cLayer, nextLayer, BCache) #need to initialize dA
end #try/catch
end #for
dAo = permutedims(dAo, [N, (1:N-1)...])
dZ = cLayer.W .* dAo
else #in case not every next layer done backprop
return Dict(:dA => Array{Any,1}(undef,0))
end #if all(i->(i.backCount==cLayer.nextLayers[1].backCount), cLayer.nextLayers)
#Z is already flipped
cLayer.dW = sum(dAo .* FCache[cLayer][:Z], dims = 1:normDim)
if regulization > 0
if regulization == 1
cLayer.dW .+= (λ / 2m)
else
cLayer.dW .+= (λ / m) .* cLayer.W
end
end
cLayer.dB = sum(dAo, dims = 1:normDim)
Num = prod(size(dAo)[1:normDim])
varep = FCache[cLayer][:var] .+ cLayer.ϵ
Ai_μ = FCache[cLayer][:Ai_μ]
Ai_μ_s = FCache[cLayer][:Ai_μ_s]
svarep = sqrt.(varep)
dZ1 = dZ ./ svarep
dZ2 = sum(dZ .* Ai_μ; dims = 1:normDim)
dZ2 .*= (-1 ./ (svarep .^ 2))
dZ2 .*= (0.5 ./ svarep)
dZ2 = dZ2 .* (1.0 / Num) .* ones(promote_type(eltype(Ai_μ), eltype(dZ2)), size(Ai_μ))
dZ2 .*= (2 .* Ai_μ)
dẐ = dZ1 .+ dZ2
dZ3 = dẐ
dZ4 = .- sum(dẐ; dims = 1:normDim)
dZ4 = dZ4 .* (1.0 / Num) .* ones(promote_type(eltype(Ai), eltype(dZ4)), size(dZ3))
dAip = dZ3 .+ dZ4
dAi = permutedims(dAip, [(2:N)..., 1])
# dẐ =
# dZ ./ sqrt.(varep) .*
# (.-(Ai_μ_s) .* ((N - 1) / N^2) ./ (varep) .^ 2 .+ 1) .*
# (1 - 1 / N)
#
# dAi = dẐ
cLayer.backCount += 1
return Dict(:dA => dAi)
end #function layerBackProp(cLayer::BatchNorm)
export layerBackProp
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 7266 | using Statistics
###Input Layer
@doc raw"""
layerForProp(
cLayer::Input,
X::AbstractArray = Array{Any,1}(undef,0);
FCache::Dict{Layer,Dict{Symbol, AbstractArray}},
kwargs...,
)
Perform forward propagation for `Input` `Layer`
# Arguments
- `cLayer` := the layer to perform for prop on
- `X` := is the input data of the `Input` `Layer`
- `FCache` := a cache holder of the for prop
# Return
- A `Dict{Symbol, AbstractArray}(:A => Ao)`
"""
function layerForProp(
cLayer::Input,
X::AbstractArray = Array{Any,1}(undef,0);
FCache::Dict{Layer,Dict{Symbol, AbstractArray}},
kwargs...,
)
if length(X) != 0
# cLayer.A = X
cLayer.inputS = cLayer.outputS = size(X)
end
cLayer.forwCount += 1
# Base.GC.gc()
return Dict(:A=>X)
end
###FCLayer forprop
@doc raw"""
layerForProp(
cLayer::FCLayer,
Ai::AbstractArray = Array{Any,1}(undef,0);
FCache::Dict{Layer,Dict{Symbol, AbstractArray}},
kwargs...,
)
Perform forward propagation for `FCLayer` `Layer`
# Arguments
- `cLayer` := the layer to perform for prop on
- `Ai` := is the input activation of the `FCLayer` `Layer`
- `FCache` := a cache holder of the for prop
# Return
- A `Dict{Symbol, AbstractArray}(:A => Ao, :Z => Z)`
"""
function layerForProp(
cLayer::FCLayer,
Ai::AbstractArray = Array{Any,1}(undef,0);
FCache::Dict{Layer,Dict{Symbol, AbstractArray}},
kwargs...,
)
prevLayer = cLayer.prevLayer
if length(Ai) == 0
Ai = FCache[prevLayer][:A]
end
cLayer.inputS = cLayer.prevLayer.outputS
Z = cLayer.W * Ai .+ cLayer.B
actFun = cLayer.actFun
# Z = cLayer.Z
cLayer.outputS = size(Z)
A = eval(:($actFun($Z)))
cLayer.forwCount += 1
# Base.GC.gc()
return Dict(:Z=>Z, :A=>A)
end #function layerForProp(cLayer::FCLayer)
###AddLayer forprop
@doc raw"""
layerForProp(
cLayer::AddLayer;
FCache::Dict{Layer,Dict{Symbol, AbstractArray}},
kwargs...,
)
Perform forward propagation for `AddLayer` `Layer`
# Arguments
- `cLayer` := the layer to perform for prop on
- `FCache` := a cache holder of the for prop
# Return
- A `Dict{Symbol, AbstractArray}(:A => Ao)`
"""
function layerForProp(
cLayer::AddLayer;
FCache::Dict{Layer,Dict{Symbol, AbstractArray}},
kwargs...,
)
A = similar(FCache[cLayer.prevLayer[1]][:A]) .= 0
# if all(
# i -> (i.forwCount == cLayer.prevLayer[1].forwCount),
# cLayer.prevLayer,
# )
for prevLayer in cLayer.prevLayer
A .+= FCache[prevLayer][:A]
end
# end #if all
cLayer.forwCount += 1
# Base.GC.gc()
return Dict(:A=>A)
end #function layerForProp(cLayer::AddLayer)
### ConcatLayer forprop
function layerForProp(
cLayer::ConcatLayer;
FCache::Dict{Layer,Dict{Symbol, AbstractArray}},
kwargs...,
)
N = ndims(FCache[cLayer.prevLayer[1]][:A])
A = cat([FCache[prevLayer][:A] for prevLayer in cLayer.prevLayer]...; dims=N-1)
cLayer.forwCount += 1
# Base.GC.gc()
return Dict(:A=>A)
end #function layerForProp(cLayer::AddLayer)
###Activation forprop
@doc raw"""
layerForProp(
cLayer::Activation,
Ai::AbstractArray = Array{Any,1}(undef,0);
FCache::Dict{Layer,Dict{Symbol, AbstractArray}},
kwargs...,
)
Perform forward propagation for `Activation` `Layer`
# Arguments
- `cLayer` := the layer to perform for prop on
- `Ai` := is the input activation of the `Activation` `Layer`
- `FCache` := a cache holder of the for prop
# Return
- A `Dict{Symbol, AbstractArray}(:A => Ao)`
"""
function layerForProp(
cLayer::Activation,
Ai::AbstractArray = Array{Any,1}(undef,0);
FCache::Dict{Layer,Dict{Symbol, AbstractArray}},
kwargs...,
)
prevLayer = cLayer.prevLayer
if length(Ai) == 0
Ai = FCache[prevLayer][:A]
end
actFun = cLayer.actFun
A = eval(:($actFun($Ai)))
cLayer.inputS = cLayer.outputS = size(Ai)
cLayer.forwCount += 1
# Ai = nothing
# Base.GC.gc()
return Dict(:A=>A)
end #function layerForProp(cLayer::Activation)
### Flatten
@doc raw"""
layerForProp(
cLayer::Flatten,
Ai::AbstractArray = Array{Any,1}(undef,0);
FCache::Dict{Layer,Dict{Symbol, AbstractArray}},
kwargs...,
)
Perform forward propagation for `Flatten` `Layer`
# Arguments
- `cLayer` := the layer to perform for prop on
- `Ai` := is the input activation of the `Flatten` `Layer`
- `FCache` := a cache holder of the for prop
# Return
- A `Dict{Symbol, AbstractArray}(:A => Ao)`
"""
function layerForProp(
cLayer::Flatten,
Ai::AbstractArray = Array{Any,1}(undef,0);
FCache::Dict{Layer,Dict{Symbol, AbstractArray}},
kwargs...,
)
prevLayer = cLayer.prevLayer
if length(Ai) == 0
Ai = FCache[prevLayer][:A]
end
cLayer.inputS = size(Ai)
cLayer.outputS = (prod(cLayer.inputS[1:end-1]), cLayer.inputS[end])
A = reshape(Ai,cLayer.outputS)
cLayer.forwCount += 1
# Ai = nothing
# Base.GC.gc()
return Dict(:A=>A)
end #function layerForProp(cLayer::Activation)
###BatchNorm
@doc raw"""
layerForProp(
cLayer::BatchNorm,
Ai::AbstractArray = Array{Any,1}(undef,0);
FCache::Dict{Layer,Dict{Symbol, AbstractArray}},
kwargs...,
)
Perform forward propagation for `BatchNorm` `Layer` and trainable parameters W and B
# Arguments
- `cLayer` := the layer to perform for prop on
- `Ai` := is the input activation of the `BatchNorm` `Layer`
- `FCache` := a cache holder of the for prop
# Return
- `Dict(
:μ => μ,
:Ai_μ => Ai_μ,
:Ai_μ_s => Ai_μ_s,
:var => var,
:Z => Z,
:A => Ao,
:Ap => Ap,
)`
"""
function layerForProp(
cLayer::BatchNorm,
Ai::AbstractArray = Array{Any,1}(undef,0);
FCache::Dict{Layer,Dict{Symbol, AbstractArray}},
kwargs...,
)
prediction = getindex(kwargs, :prediction; default=false)
prevLayer = cLayer.prevLayer
if length(Ai) == 0
Ai = FCache[prevLayer][:A]
end
if prediction
cLayer.forwCount += 1
NDim = cLayer.dim
μ = mean(Ai, dims = 1:NDim)
Ai_μ = Ai .- μ
Num = prod(size(Ai)[1:NDim])
Ai_μ_s = Ai_μ .^ 2
var = sum(Ai_μ_s, dims = 1:NDim) ./ Num
Z = Ai_μ ./ sqrt.(var .+ cLayer.ϵ)
Ao = cLayer.W .* Z .+ cLayer.B
return Dict(:A => Ao)
end #prediction
# initWB!(cLayer)
# initVS!(cLayer, model.optimizer)
N = ndims(Ai)
Ai = permutedims(Ai, [N,(1:N-1)...])
NDim = cLayer.dim + 1
μ = mean(Ai, dims = 1:NDim)
Ai_μ = Ai .- μ
Num = prod(size(Ai)[1:NDim])
Ai_μ_s = Ai_μ .^ 2
var = sum(Ai_μ_s, dims = 1:NDim) ./ Num
Z = Ai_μ ./ sqrt.(var .+ cLayer.ϵ)
Ap = cLayer.W .* Z .+ cLayer.B
Ao = permutedims(Ap, [(2:N)..., 1])
cLayer.forwCount += 1
# Ai_μ = nothing
cLayer.inputS = cLayer.outputS = size(Ao)
# Base.GC.gc()
return Dict(
:μ => μ,
:Ai_μ => Ai_μ,
:Ai_μ_s => Ai_μ_s,
:var => var,
:Z => Z,
:A => Ao,
:Ap => Ap,
)
end #function layerForProp(cLayer::BatchNorm)
export layerForProp
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 3507 | #import only the needed parts not to have conflict
import NNlib.conv, NNlib.conv!
### NNConv
function NNConv!(cLayer::Conv1D, Ai::AbstractArray{T,3}) where {T}
padS = paddingSize(cLayer, Ai)
cLayer.Z = conv(Ai, cLayer.W[end:-1:1, :, :], stride = cLayer.s, pad = padS)
Z = cLayer.Z .+= cLayer.B[:, 1, :]
actFun = cLayer.actFun
cLayer.A = eval(:($actFun($Z)))
return nothing
end #function img2colConvolve(cLayer::Conv1D
function NNConv!(cLayer::Conv2D, Ai::AbstractArray{T,4}) where {T}
padS = paddingSize(cLayer, Ai)
cLayer.Z = conv(Ai, cLayer.W[end:-1:1, end:-1:1, :, :], stride = cLayer.s, pad = padS)
Z = cLayer.Z .+= cLayer.B[:, :, 1, :]
actFun = cLayer.actFun
cLayer.A = eval(:($actFun($Z)))
return nothing
end #function img2colConvolve(cLayer::Conv2D
function NNConv!(cLayer::Conv3D, Ai::AbstractArray{T,5}) where {T}
padS = paddingSize(cLayer, Ai)
cLayer.Z = conv(
Ai,
cLayer.W[end:-1:1, end:-1:1, end:-1:1, :, :],
stride = cLayer.s,
pad = padS,
)
Z = cLayer.Z .+= cLayer.B[:, :, :, 1, :]
actFun = cLayer.actFun
cLayer.A = eval(:($actFun($Z)))
return nothing
end #function img2colConvolve(cLayer::Conv3D
export NNConv!
### dNNConv!
#import only the needed parts not to have conflict
import NNlib.∇conv_data, NNlib.∇conv_filter, NNlib.DenseConvDims
function dNNConv!(
cLayer::Conv1D,
dZ::AbstractArray{T,3},
Ai::AoN = nothing,
# Ao::AoN = nothing,
) where {AoN<:Union{AbstractArray,Nothing},T}
if Ai == nothing
Ai = cLayer.prevLayer.A
end
# if Ao == nothing
# Ao = cLayer.A
# end
W = cLayer.W
padS = paddingSize(cLayer, Ai)
convdim = DenseConvDims(Ai, W, stride = cLayer.s, padding = padS)
cLayer.dA = ∇conv_data(dZ, W[end:-1:1, :, :], convdim)
cLayer.dW[end:-1:1, :, :] = ∇conv_filter(Ai, dZ, convdim)
cLayer.dB = sum(permutedims(dZ, [1, 3, 2]), dims = 1:2)
return nothing
end #function img2colConvolve(cLayer::Conv1D
function dNNConv!(
cLayer::Conv2D,
dZ::AbstractArray,
Ai::AoN = nothing,
# Ao::AoN = nothing,
) where {AoN<:Union{AbstractArray,Nothing},T}
if Ai == nothing
Ai = cLayer.prevLayer.A
end
# if Ao == nothing
# Ao = cLayer.A
# end
W = cLayer.W
convdim = DenseConvDims(Ai, W, stride = cLayer.s)
padS = paddingSize(cLayer, Ai)
convdim = DenseConvDims(Ai, W, stride = cLayer.s, padding = padS)
cLayer.dA = ∇conv_data(dZ, W[end:-1:1, end:-1:1, :, :], convdim)
cLayer.dW[end:-1:1, end:-1:1, :, :] = ∇conv_filter(Ai, dZ, convdim)
cLayer.dB = sum(permutedims(dZ, [1, 2, 4, 3]), dims = 1:3)
return nothing
end #function img2colConvolve(cLayer::Conv2D
function dNNConv!(
cLayer::Conv3D,
dZ::AbstractArray,
Ai::AoN = nothing,
# Ao::AoN = nothing,
) where {AoN<:Union{AbstractArray,Nothing},T}
if Ai == nothing
Ai = cLayer.prevLayer.A
end
# if Ao == nothing
# Ao = cLayer.A
# end
W = cLayer.W
convdim = DenseConvDims(Ai, W, stride = cLayer.s)
padS = paddingSize(cLayer, Ai)
convdim = DenseConvDims(Ai, W, stride = cLayer.s, padding = padS)
cLayer.dA = ∇conv_data(dZ, W[end:-1:1, end:-1:1, end:-1:1, :, :], convdim)
cLayer.dW[end:-1:1, end:-1:1, end:-1:1, :, :] = ∇conv_filter(Ai, dZ, convdim)
cLayer.dB = sum(permutedims(dZ, [1, 2, 3, 5, 4]), dims = 1:4)
return nothing
end #function img2colConvolve(cLayer::Conv3D
export dNNConv!
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 24438 |
@doc raw"""
# Summary
abstract type PaddableLayer <: Layer
Abstract Type to hold all Paddable Layers (i.e. `ConvLayer` & `PoolLayer`)
# Subtypes
ConvLayer
PoolLayer
# Supertype Hierarchy
PaddableLayer <: Layer <: Any
"""
abstract type PaddableLayer <: Layer end
@doc raw"""
# Summary
abstract type ConvLayer <: PaddableLayer
Abstract Type to hold all ConvLayer
# Subtypes
Conv1D
Conv2D
Conv3D
# Supertype Hierarchy
ConvLayer <: PaddableLayer <: Layer <: Any
"""
abstract type ConvLayer <: PaddableLayer end
export ConvLayer
### Convolution layers
@doc raw"""
# Summary
mutable struct Conv2D <: ConvLayer
# Fields
channels :: Integer
f :: Tuple{Integer,Integer}
s :: Tuple{Integer,Integer}
inputS :: Tuple
outputS :: Tuple
padding :: Symbol
W :: Array{F,4} where F
dW :: Array{F,4} where F
K :: Array{F,2} where F
dK :: Array{F,2} where F
B :: Array{F,4} where F
dB :: Array{F,4} where F
actFun :: Symbol
keepProb :: AbstractFloat
V :: Dict{Symbol,Array{F,4} where F}
S :: Dict{Symbol,Array{F,4} where F}
V̂dk :: Array{F,2} where F
Ŝdk :: Array{F,2} where F
forwCount :: Integer
backCount :: Integer
updateCount :: Integer
prevLayer :: Union{Nothing, Layer}
nextLayers :: Array{Layer,1}
# Supertype Hierarchy
Conv2D <: ConvLayer <: PaddableLayer <: Layer <: Any
"""
mutable struct Conv2D <: ConvLayer
channels::Integer
"""
filter size
"""
f::Tuple{Integer, Integer}
"""
stides size
"""
s::Tuple{Integer, Integer}
inputS::Tuple
outputS::Tuple
"""
Padding mode `:valid` or `:same`
"""
padding::Symbol
W::Array{F, 4} where {F}
dW::Array{F,4} where {F}
"""
Unrolled filters
"""
K::Array{F, 2} where {F}
dK::Array{F, 2} where {F}
B::Array{F, 4} where {F}
dB::Array{F, 4} where {F}
actFun::Symbol
keepProb::AbstractFloat
# Z::Array{T,4} where {T}
#
# dA::Array{T,4} where {T}
# A::Array{T,4} where {T}
V::Dict{Symbol, Array{F, 4} where {F}}
S::Dict{Symbol, Array{F, 4} where {F}}
V̂dk::Array{F, 2} where {F}
Ŝdk::Array{F, 2} where {F}
forwCount::Integer
backCount::Integer
updateCount::Integer
prevLayer::L where {L<:Union{Layer,Nothing}}
nextLayers::Array{Layer,1}
function Conv2D(
c,
f::Tuple{Integer, Integer};
prevLayer=nothing,
strides::Tuple{Integer, Integer}=(1, 1),
padding::Symbol=:valid,
activation::Symbol=:noAct,
keepProb=1.0
)
if prevLayer == nothing
T = Any
elseif prevLayer isa Array
T = eltype(prevLayer)
else
T = eltype(prevLayer.W)
end
new(c,
f,
strides,
(0,), #inputS
(0,), #outputS
padding,
Array{T,4}(undef,0,0,0,0), #W
Array{T,4}(undef,0,0,0,0), #dW
Matrix{T}(undef,0,0), #K
Matrix{T}(undef,0,0), #dK
Array{T,4}(undef,0,0,0,0), #B
Array{T,4}(undef,0,0,0,0), #dB
activation,
keepProb,
# Array{T,4}(undef, 0,0,0,0), #Z
# Array{T,4}(undef, 0,0,0,0), #dA
# Array{T,4}(undef, 0,0,0,0), #A
Dict(:dw=>Array{T,4}(undef,0,0,0,0),
:db=>Array{T,4}(undef,0,0,0,0)), #V
Dict(:dw=>Array{T,4}(undef,0,0,0,0),
:db=>Array{T,4}(undef,0,0,0,0)), #S
Matrix{T}(undef,0,0), #V̂dk
Matrix{T}(undef,0,0), #Ŝdk
0, #forwCount
0, #backCount
0, #updateCount
prevLayer,
Array{Layer,1}(undef, 0) #nextLayer
)
end #function Conv2D
end #mutable struct Conv2D
export Conv2D
@doc raw"""
# Summary
mutable struct Conv1D <: ConvLayer
# Fields
channels :: Integer
f :: Integer
s :: Integer
inputS :: Tuple
outputS :: Tuple
padding :: Symbol
W :: Array{F,3} where F
dW :: Array{F,3} where F
K :: Array{F,2} where F
dK :: Array{F,2} where F
B :: Array{F,3} where F
dB :: Array{F,3} where F
actFun :: Symbol
keepProb :: AbstractFloat
V :: Dict{Symbol,Array{F,3} where F}
S :: Dict{Symbol,Array{F,3} where F}
V̂dk :: Array{F,2} where F
Ŝdk :: Array{F,2} where F
forwCount :: Integer
backCount :: Integer
updateCount :: Integer
prevLayer :: Union{Nothing, Layer}
nextLayers :: Array{Layer,1}
# Supertype Hierarchy
Conv1D <: ConvLayer <: PaddableLayer <: Layer <: Any
"""
mutable struct Conv1D <: ConvLayer
channels::Integer
"""
filter size
"""
f::Integer
"""
stides size
"""
s::Integer
inputS::Tuple
outputS::Tuple
padding::Symbol
W::Array{F, 3} where {F}
dW::Array{F, 3} where {F}
"""
Unrolled filters
"""
K::Array{F, 2} where {F}
dK::Array{F, 2} where {F}
B::Array{F, 3} where {F}
dB::Array{F, 3} where {F}
actFun::Symbol
keepProb::AbstractFloat
# Z::Array{T,3} where {T}
# dA::Array{T,3} where {T}
# A::Array{T,3} where {T}
V::Dict{Symbol, Array{F, 3} where {F}}
S::Dict{Symbol, Array{F, 3} where {F}}
V̂dk::Array{F, 2} where {F}
Ŝdk::Array{F, 2} where {F}
forwCount::Integer
backCount::Integer
updateCount::Integer
prevLayer::L where {L<:Union{Layer,Nothing}}
nextLayers::Array{Layer,1}
function Conv1D(c,
f::Integer;
prevLayer=nothing,
strides::Integer=1,
padding::Symbol=:valid,
activation::Symbol=:noAct,
keepProb=1.0)
if prevLayer == nothing
T = Any
elseif prevLayer isa Array
T = eltype(prevLayer)
else
T = eltype(prevLayer.W)
end
new(c,
f,
strides,
(0,), #inputS
(0,), #outputS
padding,
Array{T,3}(undef,0,0,0), #W
Array{T,3}(undef,0,0,0), #dW
Matrix{T}(undef,0,0), #K
Matrix{T}(undef,0,0), #dK
Array{T,3}(undef,0,0,0), #B
Array{T,3}(undef,0,0,0), #dB
activation,
keepProb,
# Array{T,3}(undef, 0,0,0), #Z
# Array{T,3}(undef, 0,0,0), #dA
# Array{T,3}(undef, 0,0,0), #A
Dict(:dw=>Array{T,3}(undef,0,0,0),
:db=>Array{T,3}(undef,0,0,0)), #V
Dict(:dw=>Array{T,3}(undef,0,0,0),
:db=>Array{T,3}(undef,0,0,0)), #S
Matrix{T}(undef,0,0), #V̂dk
Matrix{T}(undef,0,0), #Ŝdk
0, #forwCount
0, #backCount
0, #updateCount
prevLayer,
Array{Layer,1}(undef, 0) #nextLayer
)
end #function Conv1D
end #mutable struct Conv1D
export Conv1D
@doc raw"""
# Summary
mutable struct Conv3D <: ConvLayer
# Fields
channels :: Integer
f :: Tuple{Integer,Integer,Integer}
s :: Tuple{Integer,Integer,Integer}
inputS :: Tuple
outputS :: Tuple
padding :: Symbol
W :: Array{F,5} where F
dW :: Array{F,5} where F
K :: Array{F,2} where F
dK :: Array{F,2} where F
B :: Array{F,5} where F
dB :: Array{F,5} where F
actFun :: Symbol
keepProb :: AbstractFloat
V :: Dict{Symbol,Array{F,5} where F}
S :: Dict{Symbol,Array{F,5} where F}
V̂dk :: Array{F,2} where F
Ŝdk :: Array{F,2} where F
forwCount :: Integer
backCount :: Integer
updateCount :: Integer
prevLayer :: Union{Nothing, Layer}
nextLayers :: Array{Layer,1}
# Supertype Hierarchy
Conv3D <: ConvLayer <: PaddableLayer <: Layer <: Any
"""
mutable struct Conv3D <: ConvLayer
channels::Integer
"""
filter size
"""
f::Tuple{Integer, Integer, Integer}
"""
stides size
"""
s::Tuple{Integer, Integer, Integer}
inputS::Tuple
outputS::Tuple
padding::Symbol
W::Array{F, 5} where {F}
dW::Array{F, 5} where {F}
"""
Unrolled filters
"""
K::Array{F, 2} where {F}
dK::Array{F, 2} where {F}
B::Array{F, 5} where {F}
dB::Array{F, 5} where {F}
actFun::Symbol
keepProb::AbstractFloat
# Z::Array{T,5} where {T}
# dA::Array{T,5} where {T}
# A::Array{T,5} where {T}
V::Dict{Symbol, Array{F, 5} where {F}}
S::Dict{Symbol, Array{F, 5} where {F}}
V̂dk::Array{F, 2} where {F}
Ŝdk::Array{F, 2} where {F}
forwCount::Integer
backCount::Integer
updateCount::Integer
prevLayer::L where {L<:Union{Layer,Nothing}}
nextLayers::Array{Layer,1}
function Conv3D(c,
f::Tuple{Integer, Integer, Integer};
prevLayer=nothing,
strides::Tuple{Integer, Integer, Integer}=(1, 1, 1),
padding::Symbol=:valid,
activation::Symbol=:noAct,
keepProb=1.0)
if prevLayer == nothing
T = Any
elseif prevLayer isa Array
T = eltype(prevLayer)
else
T = eltype(prevLayer.W)
end
new(c,
f,
strides,
(0,), #inputS
(0,), #outputS
padding,
Array{T,5}(undef,0,0,0,0,0), #W
Array{T,5}(undef,0,0,0,0,0), #dW
Matrix{T}(undef,0,0), #K
Matrix{T}(undef,0,0), #dK
Array{T,5}(undef,0,0,0,0,0), #B
Array{T,5}(undef,0,0,0,0,0), #dB
activation,
keepProb,
# Array{T,5}(undef, 0,0,0,0,0), #Z
# Array{T,5}(undef, 0,0,0,0,0), #dA
# Array{T,5}(undef, 0,0,0,0,0), #A
Dict(:dw=>Array{T,5}(undef,0,0,0,0,0),
:db=>Array{T,5}(undef,0,0,0,0,0)), #V
Dict(:dw=>Array{T,5}(undef,0,0,0,0,0),
:db=>Array{T,5}(undef,0,0,0,0,0)), #S
Matrix{T}(undef,0,0), #V̂dk
Matrix{T}(undef,0,0), #Ŝdk
0, #forwCount
0, #backCount
0, #updateCount
prevLayer,
Array{Layer,1}(undef, 0) #nextLayer
)
end #function Conv3D
end #mutable struct Conv3D
export Conv3D
### Pooling layers
@doc raw"""
# Summary
abstract type PoolLayer <: PaddableLayer
Abstract Type to hold all the `PoolLayer`s
# Subtypes
AveragePoolLayer
MaxPoolLayer
# Supertype Hierarchy
PoolLayer <: PaddableLayer <: Layer <: Any
"""
abstract type PoolLayer <: PaddableLayer end
export PoolLayer
@doc raw"""
# Summary
abstract type MaxPoolLayer <: PoolLayer
Abstract Type to hold all the `MaxPoolLayer`s
# Subtypes
MaxPool1D
MaxPool2D
MaxPool3D
# Supertype Hierarchy
MaxPoolLayer <: PoolLayer <: PaddableLayer <: Layer <: Any
"""
abstract type MaxPoolLayer <: PoolLayer end
export MaxPoolLayer
@doc """
MaxPool2D(
f::Tuple{Integer,Integer}=(2,2);
prevLayer=nothing,
strides::Tuple{Integer,Integer}=f,
padding::Symbol=:valid,
)
# Summary
mutable struct MaxPool2D <: MaxPoolLayer
# Fields
channels :: Integer
f :: Tuple{Integer,Integer}
s :: Tuple{Integer,Integer}
inputS :: Tuple
outputS :: Tuple
padding :: Symbol
forwCount :: Integer
backCount :: Integer
updateCount :: Integer
prevLayer :: Union{Nothing, Layer}
nextLayers :: Array{Layer,1}
# Supertype Hierarchy
MaxPool2D <: MaxPoolLayer <: PoolLayer <: PaddableLayer <: Layer <: Any
"""
mutable struct MaxPool2D <: MaxPoolLayer
channels::Integer
"""
filter size
"""
f::Tuple{Integer, Integer}
"""
stides size
"""
s::Tuple{Integer, Integer}
inputS::Tuple
outputS::Tuple
padding::Symbol
# dA::Array{T,4} where {T}
# A::Array{T,4} where {T}
forwCount::Integer
backCount::Integer
updateCount::Integer
prevLayer::L where {L<:Union{Layer,Nothing}}
nextLayers::Array{Layer,1}
function MaxPool2D(
f::Tuple{Integer,Integer}=(2,2);
prevLayer=nothing,
strides::Tuple{Integer,Integer}=f,
padding::Symbol=:valid,
)
if prevLayer == nothing
T = Any
elseif prevLayer isa Array
T = eltype(prevLayer)
else
T = eltype(prevLayer.W)
end
return new(
0, #channels
f,
strides,
(0,), #inputS
(0,), #outputS
padding,
# Array{T,4}(undef,0,0,0,0), #dA
# Array{T,4}(undef,0,0,0,0), #A
0, #forwCount
0, #backCount
0, #updateCount
prevLayer,
Array{Layer,1}(undef,0),
)
end #function MaxPool2D
end #mutable struct MaxPool2D
export MaxPool2D
@doc """
MaxPool1D(
f::Integer=2;
prevLayer=nothing,
strides::Integer=f,
padding::Symbol=:valid,
)
# Summary
mutable struct MaxPool1D <: MaxPoolLayer
# Fields
channels :: Integer
f :: Integer
s :: Integer
inputS :: Tuple
outputS :: Tuple
padding :: Symbol
forwCount :: Integer
backCount :: Integer
updateCount :: Integer
prevLayer :: Union{Nothing, Layer}
nextLayers :: Array{Layer,1}
# Supertype Hierarchy
MaxPool1D <: MaxPoolLayer <: PoolLayer <: PaddableLayer <: Layer <: Any
"""
mutable struct MaxPool1D <: MaxPoolLayer
channels::Integer
"""
filter size
"""
f::Integer
"""
stides size
"""
s::Integer
inputS::Tuple
outputS::Tuple
padding::Symbol
# dA::Array{T,3} where {T}
# A::Array{T,3} where {T}
forwCount::Integer
backCount::Integer
updateCount::Integer
prevLayer::L where {L<:Union{Layer,Nothing}}
nextLayers::Array{Layer,1}
function MaxPool1D(
f::Integer=2;
prevLayer=nothing,
strides::Integer=f,
padding::Symbol=:valid,
)
if prevLayer == nothing
T = Any
elseif prevLayer isa Array
T = eltype(prevLayer)
else
T = eltype(prevLayer.W)
end
return new(
0, #channels
f,
strides,
(0,), #inputS
(0,), #outputS
padding,
# Array{T,3}(undef,0,0,0), #dA
# Array{T,3}(undef,0,0,0), #A
0, #forwCount
0, #backCount
0, #updateCount
prevLayer,
Array{Layer,1}(undef,0),
)
end #function MaxPool1D
end #mutable struct MaxPool1D
export MaxPool1D
@doc """
MaxPool3D(
f::Tuple{Integer,Integer,Integer}=(2,2,2);
prevLayer=nothing,
strides::Tuple{Integer,Integer,Integer}=f,
padding::Symbol=:valid,
)
# Summary
mutable struct MaxPool3D <: MaxPoolLayer
# Fields
channels :: Integer
f :: Tuple{Integer,Integer,Integer}
s :: Tuple{Integer,Integer,Integer}
inputS :: Tuple
outputS :: Tuple
padding :: Symbol
forwCount :: Integer
backCount :: Integer
updateCount :: Integer
prevLayer :: Union{Nothing, Layer}
nextLayers :: Array{Layer,1}
# Supertype Hierarchy
MaxPool3D <: MaxPoolLayer <: PoolLayer <: PaddableLayer <: Layer <: Any
"""
mutable struct MaxPool3D <: MaxPoolLayer
channels::Integer
"""
filter size
"""
f::Tuple{Integer, Integer, Integer}
"""
stides size
"""
s::Tuple{Integer, Integer, Integer}
inputS::Tuple
outputS::Tuple
padding::Symbol
# dA::Array{T,5} where {T}
# A::Array{T,5} where {T}
forwCount::Integer
backCount::Integer
updateCount::Integer
prevLayer::L where {L<:Union{Layer,Nothing}}
nextLayers::Array{Layer,1}
function MaxPool3D(
f::Tuple{Integer,Integer,Integer}=(2,2,2);
prevLayer=nothing,
strides::Tuple{Integer,Integer,Integer}=f,
padding::Symbol=:valid,
)
if prevLayer == nothing
T = Any
elseif prevLayer isa Array
T = eltype(prevLayer)
else
T = eltype(prevLayer.W)
end
return new(
0, #channels
f,
strides,
(0,), #inputS
(0,), #outputS
padding,
# Array{T,5}(undef,0,0,0,0,0), #dA
# Array{T,5}(undef,0,0,0,0,0), #A
0, #forwCount
0, #backCount
0, #updateCount
prevLayer,
Array{Layer,1}(undef,0),
)
end #function MaxPool3D
end #mutable struct MaxPool3D
export MaxPool3D
#### AveragePoolLayer
@doc raw"""
# Summary
abstract type AveragePoolLayer <: PoolLayer
# Subtypes
AveragePool1D
AveragePool2D
AveragePool3D
# Supertype Hierarchy
AveragePoolLayer <: PoolLayer <: PaddableLayer <: Layer <: Any
"""
abstract type AveragePoolLayer <: PoolLayer end
export AveragePoolLayer
@doc raw"""
AveragePool2D(
f::Tuple{Integer,Integer}=(2,2);
prevLayer=nothing,
strides::Tuple{Integer,Integer}=f,
padding::Symbol=:valid,
)
# Summary
mutable struct AveragePool2D <: AveragePoolLayer
# Fields
channels :: Integer
f :: Tuple{Integer,Integer}
s :: Tuple{Integer,Integer}
inputS :: Tuple
outputS :: Tuple
padding :: Symbol
forwCount :: Integer
backCount :: Integer
updateCount :: Integer
prevLayer :: Union{Nothing, Layer}
nextLayers :: Array{Layer,1}
# Supertype Hierarchy
AveragePool2D <: AveragePoolLayer <: PoolLayer <: PaddableLayer <: Layer <: Any
"""
mutable struct AveragePool2D <: AveragePoolLayer
channels::Integer
"""
filter size
"""
f::Tuple{Integer, Integer}
"""
stides size
"""
s::Tuple{Integer, Integer}
inputS::Tuple
outputS::Tuple
padding::Symbol
# dA::Array{T,4} where {T}
# A::Array{T,4} where {T}
forwCount::Integer
backCount::Integer
updateCount::Integer
prevLayer::L where {L<:Union{Layer,Nothing}}
nextLayers::Array{Layer,1}
function AveragePool2D(
f::Tuple{Integer,Integer}=(2,2);
prevLayer=nothing,
strides::Tuple{Integer,Integer}=f,
padding::Symbol=:valid,
)
if prevLayer == nothing
T = Any
elseif prevLayer isa Array
T = eltype(prevLayer)
else
T = eltype(prevLayer.W)
end
return new(
0, #channels
f,
strides,
(0,), #inputS
(0,), #outputS
padding,
# Array{T,4}(undef,0,0,0,0), #dA
# Array{T,4}(undef,0,0,0,0), #A
0, #forwCount
0, #backCount
0, #updateCount
prevLayer,
Array{Layer,1}(undef,0),
)
end #function AveragePool2D
end #mutable struct AveragePool2D
export AveragePool2D
@doc raw"""
AveragePool1D(
f::Integer=2;
prevLayer=nothing,
strides::Integer=f,
padding::Symbol=:valid,
)
# Summary
mutable struct AveragePool1D <: AveragePoolLayer
# Fields
channels :: Integer
f :: Integer
s :: Integer
inputS :: Tuple
outputS :: Tuple
padding :: Symbol
forwCount :: Integer
backCount :: Integer
updateCount :: Integer
prevLayer :: Union{Nothing, Layer}
nextLayers :: Array{Layer,1}
# Supertype Hierarchy
AveragePool1D <: AveragePoolLayer <: PoolLayer <: PaddableLayer <: Layer <: Any
"""
mutable struct AveragePool1D <: AveragePoolLayer
channels::Integer
"""
filter size
"""
f::Integer
"""
stides size
"""
s::Integer
inputS::Tuple
outputS::Tuple
padding::Symbol
# dA::Array{T,3} where {T}
# A::Array{T,3} where {T}
forwCount::Integer
backCount::Integer
updateCount::Integer
prevLayer::L where {L<:Union{Layer,Nothing}}
nextLayers::Array{Layer,1}
function AveragePool1D(
f::Integer=2;
prevLayer=nothing,
strides::Integer=f,
padding::Symbol=:valid,
)
if prevLayer == nothing
T = Any
elseif prevLayer isa Array
T = eltype(prevLayer)
else
T = eltype(prevLayer.W)
end
return new(
0, #channels
f,
strides,
(0,), #inputS
(0,), #outputS
padding,
# Array{T,3}(undef,0,0,0), #dA
# Array{T,3}(undef,0,0,0), #A
0, #forwCount
0, #backCount
0, #updateCount
prevLayer,
Array{Layer,1}(undef,0),
)
end #function AveragePool1D
end #mutable struct AveragePool1D
export AveragePool1D
@doc raw"""
AveragePool3D(
f::Tuple{Integer,Integer,Integer}=(2,2,2);
prevLayer=nothing,
strides::Tuple{Integer,Integer,Integer}=f,
padding::Symbol=:valid,
)
# Summary
mutable struct AveragePool3D <: AveragePoolLayer
# Fields
channels :: Integer
f :: Tuple{Integer,Integer,Integer}
s :: Tuple{Integer,Integer,Integer}
inputS :: Tuple
outputS :: Tuple
padding :: Symbol
forwCount :: Integer
backCount :: Integer
updateCount :: Integer
prevLayer :: Union{Nothing, Layer}
nextLayers :: Array{Layer,1}
# Supertype Hierarchy
AveragePool3D <: AveragePoolLayer <: PoolLayer <: PaddableLayer <: Layer <: Any
"""
mutable struct AveragePool3D <: AveragePoolLayer
channels::Integer
"""
filter size
"""
f::Tuple{Integer, Integer, Integer}
"""
stides size
"""
s::Tuple{Integer, Integer, Integer}
inputS::Tuple
outputS::Tuple
padding::Symbol
# dA::Array{T,5} where {T}
# A::Array{T,5} where {T}
forwCount::Integer
backCount::Integer
updateCount::Integer
prevLayer::L where {L<:Union{Layer,Nothing}}
nextLayers::Array{Layer,1}
function AveragePool3D(
f::Tuple{Integer,Integer,Integer}=(2,2,2);
prevLayer=nothing,
strides::Tuple{Integer,Integer,Integer}=f,
padding::Symbol=:valid,
)
if prevLayer == nothing
T = Any
elseif prevLayer isa Array
T = eltype(prevLayer)
else
T = eltype(prevLayer.W)
end
return new(
0, #channels
f,
strides,
(0,), #inputS
(0,), #outputS
padding,
# Array{T,5}(undef,0,0,0,0,0), #dA
# Array{T,5}(undef,0,0,0,0,0), #A
0, #forwCount
0, #backCount
0, #updateCount
prevLayer,
Array{Layer,1}(undef,0),
)
end #function AveragePool3D
end #mutable struct AveragePool3D
export AveragePool3D
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 4594 |
function (l::Conv1D)(li_1::Layer)
l.prevLayer = li_1
if ! in(l,li_1.nextLayers)
push!(li_1.nextLayers, l)
end
padding = l.padding
c = l.channels
n_Hi, ci, m = l.inputS = li_1.outputS
s_H = l.s
f_H = l.f
if l.padding == :same
l.outputS = (n_Hi, c, m)
elseif l.padding == :valid
n_H = ((n_Hi - f_H) ÷ s_H) + 1
l.outputS = (n_H, c, m)
end
return l
end
function (l::Conv2D)(li_1::Layer)
l.prevLayer = li_1
if ! in(l,li_1.nextLayers)
push!(li_1.nextLayers, l)
end
padding = l.padding
c = l.channels
n_Hi, n_Wi, ci, m = l.inputS = li_1.outputS
s_H, s_W = l.s
f_H, f_W = l.f
if l.padding == :same
l.outputS = (n_Hi, n_Wi, c, m)
elseif l.padding == :valid
n_H = ((n_Hi - f_H) ÷ s_H) + 1
n_W = ((n_Wi - f_W) ÷ s_W) + 1
l.outputS = (n_H, n_W, c, m)
end
return l
end
function (l::Conv3D)(li_1::Layer)
l.prevLayer = li_1
if ! in(l,li_1.nextLayers)
push!(li_1.nextLayers, l)
end
padding = l.padding
c = l.channels
n_Hi, n_Wi, n_Di, ci, m = l.inputS = li_1.outputS
s_H, s_W, s_D = l.s
f_H, f_W, f_D = l.f
if l.padding == :same
l.outputS = (n_Hi, n_Wi, n_Di, c, m)
elseif l.padding == :valid
n_H = ((n_Hi - f_H) ÷ s_H) + 1
n_W = ((n_Wi - f_W) ÷ s_W) + 1
n_D = ((n_Di - f_D) ÷ s_D) + 1
l.outputS = (n_H, n_W, n_D, c, m)
end
return l
end
function (l::MaxPool1D)(li_1::Layer)
l.prevLayer = li_1
if ! in(l,li_1.nextLayers)
push!(li_1.nextLayers, l)
end
padding = l.padding
c = l.channels = li_1.channels
n_Hi, ci, m = l.inputS = li_1.outputS
s_H = l.s
f_H = l.f
if l.padding == :same
l.outputS = (n_Hi, c, m)
elseif l.padding == :valid
n_H = ((n_Hi - f_H) ÷ s_H) + 1
l.outputS = (n_H, c, m)
end
return l
end
function (l::MaxPool2D)(li_1::Layer)
l.prevLayer = li_1
if ! in(l,li_1.nextLayers)
push!(li_1.nextLayers, l)
end
padding = l.padding
c = l.channels = li_1.channels
n_Hi, n_Wi, ci, m = l.inputS = li_1.outputS
s_H, s_W = l.s
f_H, f_W = l.f
if l.padding == :same
l.outputS = (n_Hi, n_Wi, c, m)
elseif l.padding == :valid
n_H = ((n_Hi - f_H) ÷ s_H) + 1
n_W = ((n_Wi - f_W) ÷ s_W) + 1
l.outputS = (n_H, n_W, c, m)
end
return l
end
function (l::MaxPool3D)(li_1::Layer)
l.prevLayer = li_1
if ! in(l,li_1.nextLayers)
push!(li_1.nextLayers, l)
end
padding = l.padding
c = l.channels = li_1.channels
n_Hi, n_Wi, n_Di, ci, m = l.inputS = li_1.outputS
s_H, s_W, s_D = l.s
f_H, f_W, f_D = l.f
if l.padding == :same
l.outputS = (n_Hi, n_Wi, n_Di, c, m)
elseif l.padding == :valid
n_H = ((n_Hi - f_H) ÷ s_H) + 1
n_W = ((n_Wi - f_W) ÷ s_W) + 1
n_D = ((n_Di - f_D) ÷ s_D) + 1
l.outputS = (n_H, n_W, n_D, c, m)
end
return l
end
function (l::AveragePool1D)(li_1::Layer)
l.prevLayer = li_1
if ! in(l,li_1.nextLayers)
push!(li_1.nextLayers, l)
end
padding = l.padding
c = l.channels = li_1.channels
n_Hi, ci, m = l.inputS = li_1.outputS
s_H = l.s
f_H = l.f
if l.padding == :same
l.outputS = (n_Hi, c, m)
elseif l.padding == :valid
n_H = ((n_Hi - f_H) ÷ s_H) + 1
l.outputS = (n_H, c, m)
end
return l
end
function (l::AveragePool2D)(li_1::Layer)
l.prevLayer = li_1
if ! in(l,li_1.nextLayers)
push!(li_1.nextLayers, l)
end
padding = l.padding
c = l.channels = li_1.channels
n_Hi, n_Wi, ci, m = l.inputS = li_1.outputS
s_H, s_W = l.s
f_H, f_W = l.f
if l.padding == :same
l.outputS = (n_Hi, n_Wi, c, m)
elseif l.padding == :valid
n_H = ((n_Hi - f_H) ÷ s_H) + 1
n_W = ((n_Wi - f_W) ÷ s_W) + 1
l.outputS = (n_H, n_W, c, m)
end
return l
end
function (l::AveragePool3D)(li_1::Layer)
l.prevLayer = li_1
if ! in(l,li_1.nextLayers)
push!(li_1.nextLayers, l)
end
padding = l.padding
c = l.channels = li_1.channels
n_Hi, n_Wi, n_Di, ci, m = l.inputS = li_1.outputS
s_H, s_W, s_D = l.s
f_H, f_W, f_D = l.f
if l.padding == :same
l.outputS = (n_Hi, n_Wi, n_Di, c, m)
elseif l.padding == :valid
n_H = ((n_Hi - f_H) ÷ s_H) + 1
n_W = ((n_Wi - f_W) ÷ s_W) + 1
n_D = ((n_Di - f_D) ÷ s_D) + 1
l.outputS = (n_H, n_W, n_D, c, m)
end
return l
end
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 8318 | #Naive convolution method using for loops with some parallel using
#@simd and @inbounds
function convolve!(cLayer::Conv1D, Ai::AbstractArray{T,3}) where {T}
Aip = padding(cLayer, Ai)
f_H = cLayer.f
s_H = cLayer.s
lastDim = ndims(Ai)
n_H, c, m = size(cLayer.Z)
W = cLayer.W
B = cLayer.B
@simd for mi = 1:m
@simd for ci = 1:c
@simd for hi = 1:n_H
h_start = hi * s_H - (s_H == 1 ? 0 : s_H - 1)
h_end = hi * s_H - (s_H == 1 ? 0 : s_H - 1) + f_H - 1
@inbounds ai = Aip[h_start:h_end, :, mi]
@inbounds cLayer.Z[hi, ci, mi] = W[:, :, ci] ⋅ ai
# ai = nothing
# Base.GC.gc()
end #for mi=1:m, hi=1:n_H
end #for ci=1:c
end #for mi=1:m
@inbounds cLayer.Z .+= B[:, 1, :]
Z = cLayer.Z
actFun = cLayer.actFun
cLayer.A = eval(:($actFun($Z)))
W = B = Z = nothing
Ai = nothing
# Base.GC.gc()
return nothing
end #function convolve(cLayer::Conv1D
function convolve!(cLayer::Conv2D, Ai::AbstractArray{T,4}) where {T}
Aip = padding(cLayer, Ai)
f_H, f_W = cLayer.f
s_H, s_W = cLayer.s
lastDim = ndims(Ai)
n_H, n_W, c, m = size(cLayer.Z)
W = cLayer.W
B = cLayer.B
@simd for mi = 1:m
@simd for ci = 1:c
@simd for wi = 1:n_W
@simd for hi = 1:n_H
h_start = hi * s_H - (s_H == 1 ? 0 : s_H - 1)
h_end = hi * s_H - (s_H == 1 ? 0 : s_H - 1) + f_H - 1
w_start = wi * s_W - (s_W == 1 ? 0 : s_W - 1)
w_end = wi * s_W - (s_W == 1 ? 0 : s_W - 1) + f_W - 1
@inbounds ai = Aip[h_start:h_end, w_start:w_end, :, mi]
@inbounds cLayer.Z[hi, wi, ci, mi] = W[:, :, :, ci] ⋅ ai
end #for hi=1:n_H
end #for mi=1:m, wi=1:n_W, hi=1:n_H
end #for ci=1:c
end #for mi=1:m
@inbounds cLayer.Z .+= B[:, :, 1, :]
Z = cLayer.Z
actFun = cLayer.actFun
cLayer.A = eval(:($actFun($Z)))
# Base.GC.gc()
return nothing
end #function convolve(cLayer::Conv2D
function convolve!(cLayer::Conv3D, Ai::AbstractArray{T,5}) where {T}
Aip = padding(cLayer, Ai)
f_H, f_W, f_D = cLayer.f
s_H, s_W, s_D = cLayer.s
lastDim = ndims(Ai)
n_H, n_W, n_D, c, m = size(cLayer.Z)
W = cLayer.W
B = cLayer.B
@simd for mi = 1:m
@simd for ci = 1:c
@simd for di = 1:n_D
@simd for wi = 1:n_W
@simd for hi = 1:n_H
h_start = hi * s_H - (s_H == 1 ? 0 : s_H - 1)
h_end = hi * s_H - (s_H == 1 ? 0 : s_H - 1) + f_H - 1
w_start = wi * s_W - (s_W == 1 ? 0 : s_W - 1)
w_end = wi * s_W - (s_W == 1 ? 0 : s_W - 1) + f_W - 1
d_start = di * s_D - (s_D == 1 ? 0 : s_D - 1)
d_end = di * s_D - (s_D == 1 ? 0 : s_D - 1) + f_D - 1
@inbounds ai = Aip[
h_start:h_end,
w_start:w_end,
d_start:d_end,
:,
mi,
]
@inbounds cLayer.Z[hi, wi, di, ci, mi] =
W[:, :, :, :, ci] ⋅ ai
# ai = nothing
# Base.GC.gc()
end #for hi=1:n_H
end #for wi=1:n_W
end #for di=1:n_D
end #for ci=1:c
end #for mi=1:m
@inbounds cLayer.Z .+= B[:, :, :, 1, :]
Z = cLayer.Z
actFun = cLayer.actFun
cLayer.A = eval(:($actFun($Z)))
W = B = Z = nothing
Ai = nothing
# Base.GC.gc()
return nothing
end #function convolve(cLayer::Conv3D
export convolve!
### dconvolve
#Naive convolution method using for loops with some parallel using
#@simd and @inbounds
function dconvolve!(
cLayer::Conv1D,
Ai::AbstractArray{T,3},
dAi::AbstractArray{T,3},
dZ::AbstractArray{T,3},
) where {T}
Aip = padding(cLayer, Ai)
padS = paddingSize(cLayer, Ai)
dAip = similar(Aip)
dAip .= 0
f_H = cLayer.f
s_H = cLayer.s
lastDim = ndims(Ai)
n_H, c, m = size(cLayer.Z)
W = cLayer.W
cLayer.dW = similar(W)
cLayer.dW .= 0
B = cLayer.B
cLayer.dB = similar(B)
cLayer.dB .= 0
@simd for mi = 1:m
@simd for ci = 1:c
for hi = 1:n_H
h_start = hi * s_H - (s_H == 1 ? 0 : s_H - 1)
h_end = hi * s_H - (s_H == 1 ? 0 : s_H - 1) + f_H - 1
@inbounds ai = Aip[h_start:h_end, :, mi]
@inbounds dAip[h_start:h_end, :, mi] .+=
dZ[hi, ci, mi] .* W[:, :, ci]
@inbounds cLayer.dW[:, :, ci] .+= (ai .* dZ[hi, ci, mi])
@inbounds cLayer.dB[:, :, ci] .+= dZ[hi, ci, mi]
end #for
end #for ci=1:c
end #for mi=1:m
dAi .= dAip[1+padS[1]:end-padS[2], :, :]
return dAi
end #function dconvolve(cLayer::Conv1D
function dconvolve!(
cLayer::Conv2D,
Ai::AbstractArray{T,4},
dAi::AbstractArray{T,4},
dZ::AbstractArray{T,4},
) where {T}
Aip = padding(cLayer, Ai)
padS = paddingSize(cLayer, Ai)
dAip = similar(Aip)
dAip .= 0
f_H, f_W = cLayer.f
s_H, s_W = cLayer.s
lastDim = ndims(Ai)
n_H, n_W, c, m = size(cLayer.Z)
W = cLayer.W
cLayer.dW = similar(W)
cLayer.dW .= 0
B = cLayer.B
cLayer.dB = similar(B)
cLayer.dB .= 0
@simd for mi = 1:m
@simd for ci = 1:c
for wi = 1:n_W, hi = 1:n_H
h_start = hi * s_H - (s_H == 1 ? 0 : s_H - 1)
h_end = hi * s_H - (s_H == 1 ? 0 : s_H - 1) + f_H - 1
w_start = wi * s_W - (s_W == 1 ? 0 : s_W - 1)
w_end = wi * s_W - (s_W == 1 ? 0 : s_W - 1) + f_W - 1
@inbounds ai = Aip[h_start:h_end, w_start:w_end, :, mi]
@inbounds dAip[h_start:h_end, w_start:w_end, :, mi] .+=
dZ[hi, wi, ci, mi] .* W[:, :, :, ci]
@inbounds cLayer.dW[:, :, :, ci] .+= (ai .* dZ[hi, wi, ci, mi])
@inbounds cLayer.dB[:, :, :, ci] .+= dZ[hi, wi, ci, mi]
end #for
end #for ci=1:c
end #for mi=1:m
dAi .= dAip[1+padS[1]:end-padS[2], 1+padS[3]:end-padS[4], :, :]
return dAi
end #function dconvolve(cLayer::Conv2D
function dconvolve!(
cLayer::Conv3D,
Ai::AbstractArray{T,5},
dAi::AbstractArray{T,5},
dZ::AbstractArray{T,5},
) where {T}
Aip = padding(cLayer, Ai)
padS = paddingSize(cLayer, Ai)
dAip = similar(Aip)
dAip .= 0
f_H, f_W, f_D = cLayer.f
s_H, s_W, s_D = cLayer.s
lastDim = ndims(Ai)
n_H, n_W, n_D, c, m = size(cLayer.Z)
W = cLayer.W
cLayer.dW = similar(W)
cLayer.dW .= 0
B = cLayer.B
cLayer.dB = similar(B)
cLayer.dB .= 0
@simd for mi = 1:m
@simd for ci = 1:c
for wi = 1:n_W, hi = 1:n_H, di = 1:n_D
h_start = hi * s_H - (s_H == 1 ? 0 : s_H - 1)
h_end = hi * s_H - (s_H == 1 ? 0 : s_H - 1) + f_H - 1
w_start = wi * s_W - (s_W == 1 ? 0 : s_W - 1)
w_end = wi * s_W - (s_W == 1 ? 0 : s_W - 1) + f_W - 1
d_start = di * s_D - (s_D == 1 ? 0 : s_D - 1)
d_end = di * s_D - (s_D == 1 ? 0 : s_D - 1) + f_D - 1
@inbounds ai =
Aip[h_start:h_end, w_start:w_end, d_start:d_end, :, mi]
@inbounds dAip[
h_start:h_end,
w_start:w_end,
d_start:d_end,
:,
mi,
] .+= dZ[hi, wi, di, ci, mi] .* W[:, :, :, :, ci]
@inbounds cLayer.dW[:, :, :, :, ci] .+=
(ai .* dZ[hi, wi, ci, mi])
@inbounds cLayer.dB[:, :, :, :, ci] .+= dZ[hi, wi, di, ci, mi]
end #for
end #for ci=1:c
end #for mi=1:m
dAi .= dAip[1+padS[1]:end-padS[2],
1+padS[3]:end-padS[4],
1+padS[5]:end-padS[6],
:,
:,
]
return nothing
end #function dconvolve(cLayer::Conv3D
export dconvolve!
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 548 |
export outDims
function outDims(cLayer::PL, Ai::AbstractArray{T,N}) where {PL <: PaddableLayer, N, T}
return outDims(cLayer, size(Ai))
end
function outDims(cLayer::PL, AiS::Tuple) where {PL <: PaddableLayer}
f = cLayer.f
s = cLayer.s
inputS = cLayer.inputS[1:end-2]
paddedS = paddedSize(cLayer, AiS)[1:end-2]
ci, m = cLayer.inputS[end-1:end]
co = cLayer.channels
outputS = []
for i=1:length(f)
n = (paddedS[i] - f[i]) ÷ s[i] + 1
push!(outputS, n)
end
return (outputS..., co, m)
end
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 5904 | using Statistics
function fastPooling!(
cLayer::OneD,
Ai::AbstractArray{T,3},
) where {OneD<:Union{MaxPool1D,AveragePool1D},T}
Aip = padding(cLayer, Ai)
n_Hi, c, m = size(Aip)
s_H = cLayer.s
f_H = cLayer.f
n_H = (n_Hi - f_H) ÷ s_H + 1
if cLayer isa MaxPoolLayer
cLayer.A = reshape(
maximum(reshape(Aip, f_H, n_H, c, m), dims = 1),
n_H,
c,
m,
)
else
cLayer.A =
reshape(mean(reshape(Aip, f_H, n_H, c, m), dims = 1), n_H, c, m)
end #if cLayer isa MaxPoolLayer
return nothing
end #function fastPooling!(cLayer::OneD
function fastPooling!(
cLayer::TwoD,
Ai::AbstractArray{T,4},
) where {TwoD<:Union{MaxPool2D,AveragePool2D},T}
Aip = padding(cLayer, Ai)
n_Hi, n_Wi, c, m = size(Aip)
s_H, s_W = cLayer.s
f_H, f_W = cLayer.f
n_H = (n_Hi - f_H) ÷ s_H + 1
n_W = (n_Wi - f_W) ÷ s_W + 1
if cLayer isa MaxPoolLayer
cLayer.A = reshape(
maximum(
permutedims(
reshape(Aip, f_H, n_H, f_W, n_W, c, m),
[1, 3, 2, 4, 5, 6],
),
dims = 1:2,
),
n_H,
n_W,
c,
m,
)
else
cLayer.A = reshape(
mean(
permutedims(
reshape(Aip, f_H, n_H, f_W, n_W, c, m),
[1, 3, 2, 4, 5, 6],
),
dims = 1:2,
),
n_H,
n_W,
c,
m,
)
end #if cLayer isa MaxPoolLayer
return nothing
end #function fastPooling!(cLayer::TwoD,
function fastPooling!(
cLayer::ThreeD,
Ai::AbstractArray{T,5},
) where {ThreeD<:Union{MaxPool3D,AveragePool3D},T}
Aip = padding(cLayer, Ai)
n_Hi, n_Wi, n_Di, c, m = size(Aip)
s_H, s_W, s_D = cLayer.s
f_H, f_W, f_D = cLayer.f
n_H = (n_Hi - f_H) ÷ s_H + 1
n_W = (n_Wi - f_W) ÷ s_W + 1
n_D = (n_Di - f_D) ÷ s_D + 1
if cLayer isa MaxPoolLayer
cLayer.A = reshape(
maximum(
permutedims(
reshape(Aip, f_H, n_H, f_W, n_W, f_D, n_D, c, m),
[1, 3, 5, 2, 4, 6, 7, 8],
),
dims = 1:3,
),
n_H,
n_W,
n_D,
c,
m,
)
else
cLayer.A = reshape(
mean(
permutedims(
reshape(Aip, f_H, n_H, f_W, n_W, f_D, n_D, c, m),
[1, 3, 5, 2, 4, 6, 7, 8],
),
dims = 1:3,
),
n_H,
n_W,
n_D,
c,
m,
)
end #if cLayer isa MaxPoolLayer
return nothing
end #function fastPooling!(cLayer::ThreeD,
export fastPooling!
### dfastPooling
function dfastPooling!(
cLayer::OneD,
Ai::AbstractArray{T,3},
dAi::AbstractArray{T,3},
Ao::AbstractArray{T,3},
dAo::AbstractArray{T,3},
) where {OneD<:Union{MaxPool1D,AveragePool1D},T}
padS = paddingSize(cLayer, Ai)
Aip = padding(cLayer, Ai)
n_Hi, c, m = size(Aip)
s_H = cLayer.s
f_H = cLayer.f
n_H = (n_Hi - f_H) ÷ s_H + 1
Aipr = reshape(Aip, f_H, n_H, c, m)
if cLayer isa MaxPoolLayer
mask = Aipr .== reshape(Ao, 1, n_H, c, m)
else
mask = similar(Aipr) .= 1 / prod((cLayer.f))
end #if cLayer isa MaxPoolLayer
dAi .= reshape(reshape(dAo, 1, n_H, c, m) .* mask, n_Hi, c, m)[1+padS[1]:end-padS[2], :, :]
mask = nothing
return nothing
end #function dfastPooling!(cLayer::OneD
function dfastPooling!(
cLayer::TwoD,
Ai::AbstractArray{T,4},
dAi::AbstractArray{T,4},
Ao::AbstractArray{T,4},
dAo::AbstractArray{T,4},
) where {TwoD<:Union{MaxPool2D,AveragePool2D},T}
padS = paddingSize(cLayer, Ai)
Aip = padding(cLayer, Ai)
n_Hi, n_Wi, c, m = size(Aip)
s_H, s_W = cLayer.s
f_H, f_W = cLayer.f
n_H = (n_Hi - f_H) ÷ s_H + 1
n_W = (n_Wi - f_W) ÷ s_W + 1
Aipr =
permutedims(reshape(Aip, f_H, n_H, f_W, n_W, c, m), [1, 3, 2, 4, 5, 6])
if cLayer isa MaxPoolLayer
mask = Aipr .== reshape(Ao, 1, 1, n_H, n_W, c, m)
else
mask = similar(Aipr) .= 1 / prod((cLayer.f))
end
dAi .= reshape(
permutedims(
reshape(dAo, 1, 1, n_H, n_W, c, m) .* mask,
[1, 3, 2, 4, 5, 6],
),
n_Hi,
n_Wi,
c,
m,
)[1+padS[1]:end-padS[2], 1+padS[3]:end-padS[4], :, :]
mask = nothing
return nothing
end #function dfastPooling!(cLayer::TwoD,
function dfastPooling!(
cLayer::ThreeD,
Ai::AbstractArray{T,5},
dAi::AbstractArray{T,5},
Ao::AbstractArray{T,5},
dAo::AbstractArray{T,5},
) where {ThreeD<:Union{MaxPool3D,AveragePool3D},T}
padS = paddingSize(cLayer, Ai)
Aip = padding(cLayer, Ai)
n_Hi, n_Wi, n_Di, c, m = size(Aip)
s_H, s_W, s_D = cLayer.s
f_H, f_W, f_D = cLayer.f
n_H = (n_Hi - f_H) ÷ s_H + 1
n_W = (n_Wi - f_W) ÷ s_W + 1
n_D = (n_Di - f_D) ÷ s_D + 1
Aipr = permutedims(
reshape(Aip, f_H, n_H, f_W, n_W, f_D, n_D, c, m),
[1, 3, 5, 2, 4, 6, 7, 8],
)
if cLayer isa MaxPoolLayer
mask = Aipr .== reshape(Ao, 1, 1, 1, n_H, n_W, n_D, c, m)
else
mask = similar(Aipr) .= 1 / prod((cLayer.f))
end
dAi .= reshape(
permutedims(
reshape(dAo, 1, 1, 1, n_H, n_W, n_D, c, m) .* mask,
[1, 4, 2, 5, 3, 6, 7, 8],
),
n_Hi,
n_Wi,
n_Di,
c,
m,
)[1+padS[1]:end-padS[2],
1+padS[3]:end-padS[4],
1+padS[5]:end-padS[6],
:,
:,
]
mask = nothing
return nothing
end #function dfastPooling!(cLayer::ThreeD,
export dfastPooling!
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 1754 | ###img2col
function img2col(A::AbstractArray{T,3})::AbstractArray{T,2} where {T}
S = size(A)
vs = prod(S[1:end-1])
m = S[end]
return reshape(A, vs, m)
end #function img2col(A::Array{T,3})
function img2col(A::AbstractArray{T,4})::AbstractArray{T,2} where {T}
S = size(A)
vs = prod(S[1:end-1])
m = S[end]
return reshape(permutedims(A, [2, 1, 3, 4]), vs, m)
end #function img2col(A::Array{T,4})
function img2row(A::AbstractArray{T,4})::AbstractArray{T,2} where {T}
S = size(A)
vs = prod(S[1:end-1])
m = S[end]
return reshape(A, vs, m)
end #function img2col(A::Array{T,4})
function img2col(A::AbstractArray{T,5})::AbstractArray{T,2} where {T}
S = size(A)
vs = prod(S[1:end-1])
m = S[end]
return reshape(permutedims(A, [2, 1, 3, 4, 5]), vs, m)
end #function img2col(A::Array{T,5})
export img2col
### col2img
function col2img(
Av::AbstractArray{T,2},
outputS::Tuple{Integer,Integer,Integer},
)::AbstractArray{T,3} where {T}
return reshape(Av, outputS)
end #function col2img(Av::Array{T,2}, c::Integer)
function col2img(
Av::AbstractArray{T,2},
outputS::Tuple{Integer,Integer,Integer,Integer},
)::AbstractArray{T,4} where {T}
H, W, c, m = outputS
return permutedims(reshape(Av, (W, H, c, m)), [2, 1, 3, 4])
end #function col2img(Av::Array{T,2}, c::Integer; H::Integer=-1, W::Integer=-1)
function col2img(
Av::AbstractArray{T,2},
outputS::Tuple{Integer,Integer,Integer,Integer,Integer},
)::AbstractArray{T,5} where {T}
H, W, D, c, m = outputS
return permutedims(reshape(Av, (W, H, D, c, m)), [2, 1, 3, 4, 5])
end #function col2img(Av::Array{T,2}, c::Integer; H::Integer=-1, W::Integer=-1, D::Integer=-1)
export col2img1D, col2img2D, col2img3D
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 3655 |
function img2colConvolve!(cLayer::Conv1D, Ai::AbstractArray{T,3}) where {T}
Aip = padding(cLayer, Ai)
Z =
cLayer.Z =
col2img1D(cLayer.K * img2col(Aip), cLayer.outputS) .+
cLayer.B[:, 1, :]
actFun = cLayer.actFun
cLayer.A = eval(:($actFun($Z)))
return nothing
end #function img2colConvolve(cLayer::Conv1D
function img2colConvolve!(cLayer::Conv2D, Ai::AbstractArray{T,4}) where {T}
Aip = padding(cLayer, Ai)
Z =
cLayer.Z =
col2img2D(cLayer.K * img2col(Aip), cLayer.outputS) .+
cLayer.B[:, :, 1, :]
actFun = cLayer.actFun
cLayer.A = eval(:($actFun($Z)))
return nothing
end #function img2colConvolve(cLayer::Conv2D
function img2colConvolve!(cLayer::Conv3D, Ai::AbstractArray{T,5}) where {T}
Aip = padding(cLayer, Ai)
Z =
cLayer.Z =
col2img3D(cLayer.K * img2col(Aip), cLayer.outputS) .+
cLayer.B[:, :, :, 1, :]
actFun = cLayer.actFun
cLayer.A = eval(:($actFun($Z)))
return nothing
end #function img2colConvolve(cLayer::Conv3D
export img2colConvolve!
### dimg2colConvolve!
export dimg2colConvolve!
function dimg2colConvolve!(
cLayer::Conv1D,
Ai::AbstractArray{T1,3},
dAi::AbstractArray{T2,3},
dZ::AbstractArray{T3,3},
) where {T1,T2,T3}
padS = paddingSize(cLayer, Ai)
Aip = padding(cLayer, Ai)
Aipv = img2col(Aip)
dZv = img2col(dZ)
n_Hi, c, m = size(Aip)
s_H = cLayer.s
f_H = cLayer.f
n_H = (n_Hi - f_H) ÷ s_H + 1
dAi .= col2img1D(cLayer.K' * dZv, (n_Hi,c,m))[1+padS[1]:end-padS[2], :, :]
cLayer.dK = dZv * Aipv'
cLayer.dB = sum(permutedims(dZ, [1, 3, 2]), dims = 1:2)
return nothing
end # function dimg2colConvolve!(
# cLayer::Conv1D,
# Ai::AbstractArray{T,3},
# dA::AbstractArray{T,3},
# dZ::AbstractArray{T,3},
# ) where {T}
function dimg2colConvolve!(
cLayer::Conv2D,
Ai::AbstractArray{T1,4},
dAi::AbstractArray{T2,4},
dZ::AbstractArray{T3,4},
) where {T1,T2,T3}
padS = paddingSize(cLayer, Ai)
Aip = padding(cLayer, Ai)
Aipv = img2col(Aip)
dZv = img2col(dZ)
n_Hi, n_Wi, c, m = size(Aip)
s_H, s_W = cLayer.s
f_H, f_W = cLayer.f
n_H = (n_Hi - f_H) ÷ s_H + 1
n_W = (n_Wi - f_W) ÷ s_W + 1
dAi .= col2img1D(cLayer.K' * dZv, (n_Hi,n_Wi,c,m))[1+padS[1]:end-padS[2], 1+padS[3]:end-padS[4], :, :]
cLayer.dK = dZv * Aipv'
cLayer.dB = sum(permutedims(dZ, [1, 2, 4, 3]), dims = 1:3)
return nothing
end # function dimg2colConvolve!(
# cLayer::Conv2D,
# Ai::AbstractArray{T,4},
# dA::AbstractArray{T,4},
# dZ::AbstractArray{T,4},
# ) where {T}
function dimg2colConvolve!(
cLayer::Conv3D,
Ai::AbstractArray{T1,5},
dAi::AbstractArray{T2,5},
dZ::AbstractArray{T3,5},
) where {T1,T2,T3}
padS = paddingSize(cLayer, Ai)
Aip = padding(cLayer, Ai)
Aipv = img2col(Aip)
dZv = img2col(dZ)
n_Hi, n_Wi, n_Di, c, m = size(Aip)
s_H, s_W, s_D = cLayer.s
f_H, f_W, f_D = cLayer.f
n_H = (n_Hi - f_H) ÷ s_H + 1
n_W = (n_Wi - f_W) ÷ s_W + 1
n_D = (n_Di - f_D) ÷ s_D + 1
dAi .= col2img1D(cLayer.K' * dZv, (n_Hi, n_Wi, n_Di, c, m))[1+padS[1]:end-padS[2],
1+padS[3]:end-padS[4],
1+padS[5]:end-padS[6],
:,
:,
]
cLayer.dK = dZv * Aipv'
cLayer.dB = sum(permutedims(dZ, [1, 2, 3, 5, 4]), dims = 1:4)
return nothing
end # function dimg2colConvolve!(
# cLayer::Conv3D,
# Ai::AbstractArray{T,5},
# dA::AbstractArray{T,5},
# dZ::AbstractArray{T,5},
# ) where {T}
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 463 |
include("TypeDef.jl")
include("initializations.jl")
# include("convolve.jl")
include("padding.jl")
# include("pooling.jl")
# include("layerForProp.jl")
# include("layerBackProp.jl")
include("layerUpdateParams.jl")
include("chain.jl")
include("img2col.jl")
include("unroll.jl")
include("dimensions.jl")
# include("img2colConvolve.jl")
# include("fastPooling.jl")
# include("NNConv.jl")
###
include("parallelLayerForProp.jl")
include("parallelLayerBackProp.jl")
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 4416 |
function initWB!(
cLayer::Conv1D,
p::Type{T} = Float64::Type{Float64};
He::Bool = true,
coef::AbstractFloat = 0.01,
zro::Bool = false,
) where {T}
f = cLayer.f
cl = cLayer.channels
cl_1 = cLayer.prevLayer.channels
inputS = cLayer.inputS
s_H = cLayer.s
f_H = cLayer.f
# ## note this is the input to the matmult after padding
# if cLayer.padding == :same
# p_H = Integer(ceil((s_H * (n_Hi - 1) - n_Hi + f_H) / 2))
# n_H = n_Hi + 2p_H
# elseif cLayer.padding == :valid
# n_H = n_Hi
# end
if He
coef = sqrt(2 / cl_1)
end
if zro
W = zeros(T, f..., cl_1, cl)
else
W = T.(randn(f..., cl_1, cl) .* coef)
end
B = zeros(T, repeat([1], length(f) + 1)..., cl)
cLayer.W, cLayer.B = W, B
cLayer.K = Matrix{T}(undef,0,0)
#unroll(cLayer, (n_H, ci, m))
cLayer.dW = zeros(T, f..., cl_1, cl)
cLayer.dK = Matrix{T}(undef,0,0)
#unroll(cLayer, (n_H, ci, m), :dW)
cLayer.dB = deepcopy(B)
return nothing
end #initWB
function initWB!(
cLayer::Conv2D,
p::Type{T} = Float64::Type{Float64};
He::Bool = true,
coef::AbstractFloat = 0.01,
zro::Bool = false,
) where {T}
f = cLayer.f
cl = cLayer.channels
cl_1 = cLayer.prevLayer.channels
inputS = cLayer.inputS
s_H, s_W = cLayer.s
f_H, f_W = cLayer.f
## note this is the input to the matmult after padding
# if cLayer.padding == :same
# p_H = Integer(ceil((s_H * (n_Hi - 1) - n_Hi + f_H) / 2))
# p_W = Integer(ceil((s_W * (n_Wi - 1) - n_Wi + f_W) / 2))
# n_H, n_W = n_Hi + 2p_H, n_Wi + 2p_W
# elseif cLayer.padding == :valid
# n_H = n_Hi
# n_W = n_Wi
# end
if He
coef = sqrt(2 / cl_1)
end
if zro
W = zeros(T, f..., cl_1, cl)
else
W = T.(randn(f..., cl_1, cl) .* coef)
end
B = zeros(T, repeat([1], length(f) + 1)..., cl)
cLayer.W, cLayer.B = W, B
cLayer.K = Matrix{T}(undef,0,0)
#unroll(cLayer, (n_H, n_W, ci, m))
cLayer.dW = zeros(T, f..., cl_1, cl)
cLayer.dK = Matrix{T}(undef,0,0)
#unroll(cLayer, (n_H, n_W, ci, m), :dW)
cLayer.dB = deepcopy(B)
return nothing
end #initWB
function initWB!(
cLayer::Conv3D,
p::Type{T} = Float64::Type{Float64};
He::Bool= true,
coef::AbstractFloat = 0.01,
zro::Bool = false,
) where {T}
f = cLayer.f
cl = cLayer.channels
cl_1 = cLayer.prevLayer.channels
inputS = cLayer.inputS
s_H, s_W, s_D = cLayer.s
f_H, f_W, f_D = cLayer.f
## note this is the input to the matmult after padding
# if cLayer.padding == :same
# p_H = Integer(ceil((s_H * (n_Hi - 1) - n_Hi + f_H) / 2))
# p_W = Integer(ceil((s_W * (n_Wi - 1) - n_Wi + f_W) / 2))
# p_D = Integer(ceil((s_D * (n_Di - 1) - n_Di + f_D) / 2))
# n_H, n_W, n_D = n_Hi + 2p_H, n_Wi + 2p_W, n_Di + 2p_D
# elseif cLayer.padding == :valid
# n_H = n_Hi
# n_W = n_Wi
# n_D = n_Di
# end
if He
coef = sqrt(2 / cl_1)
end
if zro
W = zeros(T, f..., cl_1, cl)
else
W = T.(randn(f..., cl_1, cl) .* coef)
end
B = zeros(T, repeat([1], length(f) + 1)..., cl)
cLayer.W, cLayer.B = W, B
cLayer.K = Matrix{T}(undef,0,0)
#unroll(cLayer, (n_H, n_W, n_D, ci, m))
cLayer.dW = zeros(T, f..., cl_1, cl)
cLayer.dK = Matrix{T}(undef,0,0)
#unroll(cLayer, (n_H, n_W, n_D, ci, m), :dW)
cLayer.dB = deepcopy(B)
return nothing
end #initWB
###Pooling Layers
function initWB!(
cLayer::P,
p::Type{T} = Float64::Type{Float64};
He::Bool = true,
coef::AbstractFloat = 0.01,
zro::Bool = false,
) where {T,P<:PoolLayer}
return nothing
end
export initWB!
### initVS!
function initVS!(cLayer::CL, optimizer::Symbol) where {CL <: ConvLayer}
if optimizer == :adam || optimizer == :momentum
cLayer.V[:dw] = deepcopy(cLayer.dW)
cLayer.V̂dk = deepcopy(cLayer.dK)
cLayer.V[:db] = deepcopy(cLayer.dB)
if optimizer == :adam
cLayer.S = deepcopy(cLayer.V)
cLayer.Ŝdk = deepcopy(cLayer.V̂dk)
end #if optimizer == :adam
end #if optimizer == :adam || optimizer == :momentum
return nothing
end #function initVS!
initVS!(cLayer::P, optimizer::Symbol) where {P<:PoolLayer} = nothing
export initVS!
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 7884 |
### convlayers
function layerBackProp(
cLayer::ConvLayer,
model::Model,
actFun::SoS,
labels::AbstractArray,
) where {SoS<:Union{Type{softmax},Type{σ}}}
lossFun = model.lossFun
dlossFun = Symbol("d", lossFun)
A = cLayer.A
Y = labels
dZ = eval(:($dlossFun($A, $Y)))
return dZ
end #softmax or σ layerBackProp
function layerBackProp!(
cLayer::CL,
model::Model,
Ai::AbstractArray = Array{Any,1}(undef,0),
Ao::AbstractArray = Array{Any,1}(undef,0),
dAo::AbstractArray = Array{Any,1}(undef,0);
labels::AbstractArray = Array{Any,1}(undef,0),
kwargs...
) where {CL <: ConvLayer}
NNlib = getindex(kwargs, :NNlib; default=true)
img2col = getindex(kwargs, :img2col; default=true)
if length(Ai) == 0
Ai = cLayer.prevLayer.A
end
if length(Ao) == 0
Ao = cLayer.A
end
m = size(Ao)[end]
regulization, λ = model.regulization, model.λ
actFun = cLayer.actFun
dZ = []
if cLayer.actFun == model.outLayer.actFun
dZ = layerBackProp(cLayer, model, eval(:($actFun)), labels)
elseif length(dAo) != 0
dActFun = Symbol("d", cLayer.actFun)
Z = cLayer.Z
dZ = dAo .* eval(:($dActFun($Z)))
elseif all(
i -> (i.backCount == cLayer.nextLayers[1].backCount),
cLayer.nextLayers,
)
dAo = []
for nextLayer in cLayer.nextLayers
try
dAo .+= nextLayer.dA
catch e
dAo = nextLayer.dA #need to initialize dA
end #try/catch
end #for
dActFun = Symbol("d", cLayer.actFun)
Z = cLayer.Z
dZ = dAo .* eval(:($dActFun($Z)))
else #in case not every next layer done backprop
return nothing
end #if all(i->(i.backCount==cLayer.nextLayers[1].backCount), cLayer.nextLayers)
if NNlib
dNNConv!(cLayer, dZ, Ai)
else
cLayer.dA = similar(Ai) #the size before padding
cLayer.dA .= 0
if img2col
dimg2colConvolve!(cLayer, Ai, cLayer.dA, dZ)
else
dconvolve!(cLayer, Ai, cLayer.dA, dZ)
end #if img2col
end #if NNlib
cLayer.backCount += 1
return nothing
end #function layerBackProp!(cLayer::Input
### Pooling Layers
#import only the needed parts not to have conflict
import NNlib.∇maxpool, NNlib.∇meanpool, NNlib.∇maxpool!, NNlib.∇meanpool!, NNlib.PoolDims
function layerBackProp!(
cLayer::OneD,
model::Model,
Ai::AbstractArray = Array{Any,1}(undef,0),
Ao::AbstractArray = Array{Any,1}(undef,0),
dAo::AbstractArray = Array{Any,1}(undef,0);
labels::AbstractArray = Array{Any,1}(undef,0),
kwargs...
) where {OneD<:Union{MaxPool1D,AveragePool1D}}
NNlib = getindex(kwargs, :NNlib) ? kwargs[:NNlib] : true
if length(Ai) == 0
Ai = cLayer.prevLayer.A
end
if length(Ao) == 0
Ao = cLayer.A
end
padS = paddingSize(cLayer, Ai)
cLayer.dA = similar(Ai) .= 0
if length(dAo) == 0
if all(
i -> (i.backCount == cLayer.nextLayers[1].backCount),
cLayer.nextLayers,
)
dAo = []
for nextLayer in cLayer.nextLayers
try
dAo .+= nextLayer.dA
catch e
dAo = nextLayer.dA #need to initialize dA
end #try/catch
end #for
else
return nothing
end #if all(i->(i.backCount==cLayer.nextLayers[1].backCount), cLayer.nextLayers)
end #if dA==nothing
if NNlib
pooldims = PoolDims(Ai, cLayer.f, stride = cLayer.s, padding = padS)
if cLayer isa MaxPoolLayer
∇maxpool!(cLayer.dA, dAo, Ao, Ai, pooldims)
elseif cLayer isa AveragePoolLayer
∇meanpool!(cLayer.dA, dAo, Ao, Ai, pooldims)
end #if cLayer isa MaxPoolLayer
else
if cLayer.s == cLayer.f
dfastPooling!(cLayer, Ai, cLayer.dA, Ao, dAo)
else
dpooling!(cLayer, Ai, cLayer.dA, Ao, dAo)
end #if cLayer.s == cLayer.f
end #if NNlib
cLayer.backCount += 1
return nothing
end #unction layerBackProp!(cLayer::OneD) where {OneD <: Union{MaxPool1D, AveragePool1D}}
function layerBackProp!(
cLayer::TwoD,
model::Model,
Ai::AbstractArray = Array{Any,1}(undef,0),
Ao::AbstractArray = Array{Any,1}(undef,0),
dAo::AbstractArray = Array{Any,1}(undef,0);
labels::AbstractArray = Array{Any,1}(undef,0),
kwargs...
) where {TwoD<:Union{MaxPool2D,AveragePool2D}}
NNlib = getindex(kwargs, :NNlib) ? kwargs[:NNlib] : true
if length(Ai) == 0
Ai = cLayer.prevLayer.A
end
if length(Ao) == 0
Ao = cLayer.A
end
padS = paddingSize(cLayer, Ai)
cLayer.dA = similar(Ai) .= 0
if length(dAo) == 0
if all(
i -> (i.backCount == cLayer.nextLayers[1].backCount),
cLayer.nextLayers,
)
dAo = []
for nextLayer in cLayer.nextLayers
try
dAo .+= nextLayer.dA
catch e
dAo = nextLayer.dA #need to initialize dA
end #try/catch
end #for
else
return nothing
end #if all(i->(i.backCount==cLayer.nextLayers[1].backCount), cLayer.nextLayers)
end #if dA==nothing
if NNlib
pooldims = PoolDims(Ai, cLayer.f, stride = cLayer.s, padding = padS)
if cLayer isa MaxPoolLayer
∇maxpool!(cLayer.dA, dAo, Ao, Ai, pooldims)
elseif cLayer isa AveragePoolLayer
∇meanpool!(cLayer.dA, dAo, Ao, Ai, pooldims)
end #if cLayer isa MaxPoolLayer
else
if cLayer.s == cLayer.f
dfastPooling!(cLayer, Ai, cLayer.dA, Ao, dAo)
else
dpooling!(cLayer, Ai, cLayer.dA, Ao, dAo)
end #if cLayer.s == cLayer.f
end #if NNlib
cLayer.backCount += 1
return nothing
end #function layerBackProp!(cLayer::TwoD) where {TwoD <: Union{MaxPool2D, AveragePool2D}}
function layerBackProp!(
cLayer::ThreeD,
model::Model,
Ai::AbstractArray = Array{Any,1}(undef,0),
Ao::AbstractArray = Array{Any,1}(undef,0),
dAo::AbstractArray = Array{Any,1}(undef,0);
labels::AbstractArray = Array{Any,1}(undef,0),
kwargs...,
) where {ThreeD<:Union{MaxPool3D,AveragePool3D}}
NNlib = getindex(kwargs, :NNlib) ? kwargs[:NNlib] : true
if length(Ai) == 0
Ai = cLayer.prevLayer.A
end
if length(Ao) == 0
Ao = cLayer.A
end
padS = paddingSize(cLayer, Ai)
cLayer.dA = similar(Ai) .= 0
if length(dAo) == 0
if all(
i -> (i.backCount == cLayer.nextLayers[1].backCount),
cLayer.nextLayers,
)
dAo = []
for nextLayer in cLayer.nextLayers
try
dAo .+= nextLayer.dA
catch e
dAo = nextLayer.dA #need to initialize dA
end #try/catch
end #for
else
return nothing
end #if all(i->(i.backCount==cLayer.nextLayers[1].backCount), cLayer.nextLayers)
end #if dA==nothing
if NNlib
pooldims = PoolDims(Ai, cLayer.f, stride = cLayer.s, padding = padS)
if cLayer isa MaxPoolLayer
∇maxpool!(cLayer.dA, dAo, Ao, Ai, pooldims)
elseif cLayer isa AveragePoolLayer
∇meanpool!(cLayer.dA, dAo, Ao, Ai, pooldims)
end #if cLayer isa MaxPoolLayer
else
if cLayer.s == cLayer.f
dfastPooling!(cLayer, Ai, cLayer.dA, Ao, dAo)
else
dpooling!(cLayer, Ai, cLayer.dA, Ao, dAo)
end #if cLayer.s == cLayer.f
end #if NNlib
cLayer.backCount += 1
return nothing
end #function layerBackProp!(cLayer::ThreeD) where {ThreeD <: Union{MaxPool3D, AveragePool3D}}
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 7575 |
###convolution layers forprop
function layerForProp!(
cLayer::Conv1D,
Ai::AbstractArray = Array{Any,1}(undef,0);
kwargs...
)
NNlib = getindex(kwargs, :NNlib) ? kwargs[:NNlib] : true
img2col = getindex(kwargs, :img2col) ? kwargs[:img2col] : false
if length(Ai) == 0
Ai = cLayer.prevLayer.A
end
cLayer.inputS = size(Ai)
# Ai = padding(cLayer, Ai)
n_Hi, ci, m = paddedSize(cLayer, Ai)
s_H = cLayer.s
f_H = cLayer.f
c = cLayer.channels
n_H = (n_Hi - f_H) ÷ s_H + 1
if NNlib
NNConv!(cLayer, Ai)
elseif img2col
## in case input different size than previous time
if (n_H * c, n_Hi * ci) != size(cLayer.K)
cLayer.K = unroll(cLayer, (n_Hi, ci, m))
end
img2colConvolve!(cLayer, Ai)
else
cLayer.Z = zeros(eltype(Ai), n_H, cLayer.channels, m)
convolve!(cLayer, Ai)
end
cLayer.outputS = size(cLayer.A)
Ai = nothing
cLayer.forwCount += 1
# Base.GC.gc()
return nothing
end #function layerForProp!(cLayer::Conv1D)
function layerForProp!(
cLayer::Conv2D,
Ai::AbstractArray = Array{Any,1}(undef,0);
kwargs...
)
NNlib = getindex(kwargs, :NNlib) ? kwargs[:NNlib] : true
img2col = getindex(kwargs, :img2col) ? kwargs[:img2col] : false
if length(Ai) == 0
Ai = cLayer.prevLayer.A
end
cLayer.inputS = size(Ai)
# Ai = padding(cLayer, Ai)
n_Hi, n_Wi, ci, m = paddedSize(cLayer, Ai)
c = cLayer.channels
s_H, s_W = cLayer.s
f_H, f_W = cLayer.f
c = cLayer.channels
n_H = (n_Hi - f_H) ÷ s_H + 1
n_W = (n_Wi - f_W) ÷ s_W + 1
if NNlib
NNConv!(cLayer, Ai)
elseif img2col
## in case input different size than previous time
if (n_W * n_H * c, n_Hi * n_Wi * ci) != size(cLayer.K)
cLayer.K = unroll(cLayer, (n_Hi, n_Wi, ci, m))
end #if (n_W*n_H* c, n_Hi*n_Wi*ci) != size(cLayer.K)
img2colConvolve!(cLayer, Ai)
else
cLayer.Z = zeros(eltype(Ai), n_H, n_W, cLayer.channels, m)
convolve!(cLayer, Ai)
end #if img2colConvolve
cLayer.outputS = size(cLayer.A)
Ai = nothing
cLayer.forwCount += 1
# Base.GC.gc()
return nothing
end #function layerForProp!(cLayer::Conv2D)
function layerForProp!(
cLayer::Conv3D,
Ai::AbstractArray = Array{Any,1}(undef,0);
kwargs...
)
NNlib = getindex(kwargs, :NNlib) ? kwargs[:NNlib] : true
img2col = getindex(kwargs, :img2col) ? kwargs[:img2col] : false
if length(Ai) == 0
Ai = cLayer.prevLayer.A
end
cLayer.inputS = size(Ai)
# Ai = padding(cLayer, Ai)
n_Hi, n_Wi, n_Di, ci, m = paddedSize(cLayer, Ai)
s_H, s_W, s_D = cLayer.s
f_H, f_W, f_D = cLayer.f
c = cLayer.channels
n_H = (n_Hi - f_H) ÷ s_H + 1
n_W = (n_Wi - f_W) ÷ s_W + 1
n_D = (n_Di - f_D) ÷ s_D + 1
if NNlib
NNConv!(cLayer, Ai)
elseif img2col
## in case input different size than previous time
if (n_W * n_H * n_D * c, n_Hi * n_Wi * n_Di * ci) != size(cLayer.K)
cLayer.K = unroll(cLayer, (n_Hi, n_Wi, n_Di, ci, m))
end #if (n_W*n_H* c, n_Hi*n_Wi*ci) != size(cLayer.K)
img2colConvolve!(cLayer, Ai)
else
cLayer.Z = zeros(eltype(Ai), n_H, n_W, n_D, cLayer.channels, m)
convolve!(cLayer, Ai)
end #if img2colConvolve
cLayer.outputS = size(cLayer.A)
cLayer.forwCount += 1
Ai = nothing
# Base.GC.gc()
return nothing
end #function layerForProp!(cLayer::Conv3D)
### Pooling Layers
#import only the needed parts not to have conflict
import NNlib.maxpool, NNlib.meanpool, NNlib.maxpool!, NNlib.meanpool!, NNlib.PoolDims
function layerForProp!(
cLayer::OneD,
Ai::AbstractArray = Array{Any,1}(undef,0);
kwargs...
) where {OneD<:Union{MaxPool1D,AveragePool1D}}
NNlib = getindex(kwargs, :NNlib) ? kwargs[:NNlib] : true
if length(Ai) == 0
Ai = cLayer.prevLayer.A
end
cLayer.inputS = size(Ai)
# Ai = padding(cLayer, Ai)
padS = paddingSize(cLayer, Ai)
n_Hi, ci, m = paddedSize(cLayer, Ai)
s_H = cLayer.s
f_H = cLayer.f
c = cLayer.channels
n_H = (n_Hi - f_H) ÷ s_H + 1
cLayer.A = zeros(eltype(Ai), n_H, c, m)
if NNlib
pooldims = PoolDims(Ai, cLayer.f, stride = cLayer.s, padding = padS)
if cLayer isa MaxPoolLayer
maxpool!(cLayer.A, Ai, pooldims)
elseif cLayer isa AveragePoolLayer
meanpool!(cLayer.A, Ai, pooldims)
end #if cLayer isa MaxPoolLayer
elseif f_H == s_H #to use the built-in reshape and maximum and mean
fastPooling!(cLayer, Ai)
else
pooling!(cLayer, Ai)
end #if NNlibConv
cLayer.outputS = size(cLayer.A)
Ai = nothing
cLayer.forwCount += 1
# Base.GC.gc()
return nothing
end #unction layerForProp!(cLayer::OneD) where {OneD <: Union{MaxPool1D, AveragePool1D}}
function layerForProp!(
cLayer::TwoD,
Ai::AbstractArray = Array{Any,1}(undef,0);
kwargs...,
) where {TwoD<:Union{MaxPool2D,AveragePool2D}}
NNlib = getindex(kwargs, :NNlib) ? kwargs[:NNlib] : true
if length(Ai) == 0
Ai = cLayer.prevLayer.A
end
cLayer.inputS = size(Ai)
# Ai = padding(cLayer, Ai)
padS = paddingSize(cLayer, Ai)
n_Hi, n_Wi, ci, m = paddedSize(cLayer, Ai)
s_H, s_W = S = cLayer.s
f_H, f_W = F = cLayer.f
c = cLayer.channels
n_H = (n_Hi - f_H) ÷ s_H + 1
n_W = (n_Wi - f_W) ÷ s_W + 1
cLayer.A =
zeros(eltype(Ai), n_H, n_W, c, m)
if NNlib
pooldims = PoolDims(Ai, cLayer.f, stride = cLayer.s, padding = padS)
if cLayer isa MaxPoolLayer
maxpool!(cLayer.A, Ai, pooldims)
elseif cLayer isa AveragePoolLayer
meanpool!(cLayer.A, Ai, pooldims)
end #if cLayer isa MaxPoolLayer
elseif F == S #to use the built-in reshape, maximum and mean
fastPooling!(cLayer, Ai)
else
pooling!(cLayer, Ai)
end #if NNlibConv
cLayer.outputS = size(cLayer.A)
Ai = nothing
cLayer.forwCount += 1
# Base.GC.gc()
return nothing
end #function layerForProp!(cLayer::TwoD) where {TwoD <: Union{MaxPool2D, AveragePool2D}}
function layerForProp!(
cLayer::ThreeD,
Ai::AbstractArray = Array{Any,1}(undef,0);
kwargs...,
) where {ThreeD<:Union{MaxPool3D,AveragePool3D}}
NNlib = getindex(kwargs, :NNlib) ? kwargs[:NNlib] : true
if length(Ai) == 0
Ai = cLayer.prevLayer.A
end
cLayer.inputS = size(Ai)
# Ai = padding(cLayer, Ai)
padS = paddingSize(cLayer, Ai)
n_Hi, n_Wi, n_Di, ci, m = paddedSize(cLayer, Ai)
s_H, s_W, s_D = S = cLayer.s
f_H, f_W, f_D = F = cLayer.f
c = cLayer.channels
n_H = (n_Hi - f_H) ÷ s_H + 1
n_W = (n_Wi - f_W) ÷ s_W + 1
n_D = (n_Di - f_D) ÷ s_D + 1
cLayer.A =
zeros(eltype(Ai), n_H, n_W, n_D, c, m)
if NNlib
pooldims = PoolDims(Ai, cLayer.f, stride = cLayer.s, padding = padS)
if cLayer isa MaxPoolLayer
maxpool!(cLayer.A, Ai, pooldims)
elseif cLayer isa AveragePoolLayer
meanpool!(cLayer.A, Ai, pooldims)
end #if cLayer isa MaxPoolLayer
elseif F == S #to use the built-in reshape and maximum and mean
fastPooling!(cLayer, Ai)
else
pooling!(cLayer, Ai)
end #if NNlibConv
cLayer.outputS = size(cLayer.A)
Ai = nothing
cLayer.forwCount += 1
# Base.GC.gc()
return nothing
end #function layerForProp!(cLayer::ThreeD) where {ThreeD <: Union{MaxPool3D, AveragePool3D}}
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 2983 |
#TODO make sure to renew V and S at each epoch
function layerUpdateParams!(
model::Model,
cLayer::CL,
cnt::Integer = -1;
tMiniBatch::Integer = 1,
kwargs...,
) where {CL<:ConvLayer}
optimizer = model.optimizer
α = model.α
β1, β2, ϵAdam = model.β1, model.β2, model.ϵAdam
if cLayer.updateCount >= cnt
return nothing
end #if cLayer.updateCount >= cnt
if !all(
i -> (i.updateCount == cLayer.nextLayers[1].updateCount),
cLayer.nextLayers,
)
return nothing
end
#initialize the needed variables to hold the corrected values
#it is being init here cause these are not needed elsewhere
if optimizer == :adam || optimizer == :momentum
VCorrected = Dict(:dw => similar(cLayer.dW), :db => similar(cLayer.dB))
if tMiniBatch == 1
cLayer.V[:dw] .= 0
cLayer.V[:db] .= 0
end
cLayer.V[:dw] .= β1 .* cLayer.V[:dw] .+ (1 - β1) .* cLayer.dW
cLayer.V[:db] .= β1 .* cLayer.V[:db] .+ (1 - β1) .* cLayer.dB
##correcting
VCorrected[:dw] .= cLayer.V[:dw] ./ (1 - β1^tMiniBatch)
VCorrected[:db] .= cLayer.V[:db] ./ (1 - β1^tMiniBatch)
if optimizer == :adam
SCorrected =
Dict(:dw => similar(cLayer.dW), :db => similar(cLayer.dB))
if tMiniBatch == 1
cLayer.S[:dw] .= 0
cLayer.S[:db] .= 0
end
cLayer.S[:dw] .= β2 .* cLayer.S[:dw] .+ (1 - β2) .* (cLayer.dW .^ 2)
cLayer.S[:db] .= β2 .* cLayer.S[:db] .+ (1 - β2) .* (cLayer.dB .^ 2)
##correcting
SCorrected[:dw] .= cLayer.S[:dw] ./ (1 - β2^tMiniBatch)
SCorrected[:db] .= cLayer.S[:db] ./ (1 - β2^tMiniBatch)
##update parameters with adam
cLayer.W .-=
(α .* (VCorrected[:dw] ./ (sqrt.(SCorrected[:dw]) .+ ϵAdam)))
cLayer.B .-=
(α .* (VCorrected[:db] ./ (sqrt.(SCorrected[:db]) .+ ϵAdam)))
VCorrected = nothing
SCorrected = nothing
else#if optimizer==:momentum
cLayer.W .-= (α .* VCorrected[:dw])
cLayer.B .-= (α .* VCorrected[:db])
VCorrected = nothing
end #if optimizer==:adam
else
cLayer.W .-= (α .* cLayer.dW)
cLayer.B .-= (α .* cLayer.dB)
end #if optimizer==:adam || optimizer==:momentum
cLayer.updateCount += 1
# Base.GC.gc()
return nothing
end #layerUpdateParams!
function layerUpdateParams!(
model::Model,
cLayer::PL,
cnt::Integer = -1;
tMiniBatch::Integer = 1,
kwargs...,
) where {PL<:PoolLayer}
if cLayer.updateCount >= cnt
return nothing
end #if cLayer.updateCount >= cnt
if !all(
i -> (i.updateCount == cLayer.nextLayers[1].updateCount),
cLayer.nextLayers,
)
return nothing
end
cLayer.updateCount += 1
return nothing
end #updateParams!
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 5254 | using PaddedViews
###
export paddedSize
function paddedSize(cLayer::PL, Ai::AoN=nothing) where {PL<:PaddableLayer, AoN <: Union{AbstractArray, Nothing}}
if Ai == nothing
Ai = cLayer.prevLayer.A
end
return paddedSize(cLayer, size(Ai))
end #function paddedSize(cLayer::PL, Ai::AoN=nothing) where {PL<:PaddableLayer, AoN <: Union{AbstractArray, Nothing}}
function paddedSize(cLayer::PL, AiS::Tuple) where {PL<:PaddableLayer}
padS = paddingSize(cLayer, AiS)
outPS = [i for i in AiS]
for i=1:(length(AiS)-2)
outPS[i] += padS[2i-1] + padS[2i]
end
return Tuple(outPS)
end #function paddedSize(cLayer::PL, AiS::Tuple) where {PL<:PaddableLayer}
###
export paddingSize
function paddingSize(cLayer::PL, Ai::AoN=nothing) where {PL<:PaddableLayer, AoN <: Union{AbstractArray, Nothing}}
if Ai == nothing
Ai = cLayer.prevLayer.A
end
return paddingSize(cLayer, size(Ai))
end
"""
function paddingSize(cLayer::PL, Ai::AbstractArray) where {PL<:PaddableLayer}
Helping function that returns the p_H_hi, p_H_lo, and (in case 2D Conv), p_W_hi, p_W_lo, and so on
"""
function paddingSize(cLayer::PL, AiS::Tuple) where {PL<:PaddableLayer}
ndim = length(AiS)
if cLayer.padding == :valid
return Tuple(repeat([0], (ndim-2)*2))
end
if ndim == 3
n_Hi, ci, m = AiS
s_H = cLayer.s
f_H = cLayer.f
if cLayer.padding == :same
p_H = s_H * (n_Hi - 1) - n_Hi + f_H
p_H_hi = p_H ÷ 2 + ((p_H % 2 == 0) ? 0 : 1)
p_H_lo = p_H ÷ 2
n_H = n_Hi
return (p_H_hi, p_H_lo)
end
elseif ndim == 4
n_Hi, n_Wi, ci, m = AiS
(s_H, s_W) = cLayer.s
(f_H, f_W) = cLayer.f
if cLayer.padding == :same
p_H = (s_H * (n_Hi - 1) - n_Hi + f_H)
p_W = (s_W * (n_Wi - 1) - n_Wi + f_W)
p_H_hi = p_H ÷ 2 + ((p_H % 2 == 0) ? 0 : 1)
p_H_lo = p_H ÷ 2
p_W_hi = p_W ÷ 2 + ((p_W % 2 == 0) ? 0 : 1)
p_W_lo = p_W ÷ 2
n_H, n_W = n_Hi, n_Wi
return (p_H_hi, p_H_lo, p_W_hi, p_W_lo)
end
elseif ndim == 5
n_Hi, n_Wi, n_Di, ci, m = AiS
s_H, s_W, s_D = cLayer.s
f_H, f_W, f_D = cLayer.f
if cLayer.padding == :same
p_H = (s_H * (n_Hi - 1) - n_Hi + f_H)
p_W = (s_W * (n_Wi - 1) - n_Wi + f_W)
p_D = (s_D * (n_Di - 1) - n_Di + f_D)
p_H_hi = p_H ÷ 2 + ((p_H % 2 == 0) ? 0 : 1)
p_H_lo = p_H ÷ 2
p_W_hi = p_W ÷ 2 + ((p_W % 2 == 0) ? 0 : 1)
p_W_lo = p_W ÷ 2
p_D_hi = p_D ÷ 2 + ((p_D % 2 == 0) ? 0 : 1)
p_D_lo = p_D ÷ 2
n_H, n_W, n_D = n_Hi, n_Wi, n_Di
return (p_H_hi, p_H_lo, p_W_hi, p_W_lo, p_D_hi, p_D_lo)
end #if cLayer.padding == :same
end
end #function paddingSize(cLayer::CL, AiS::Tuple)
###Padding functions
function padding(
cLayer::P,
Ai::AoN = nothing,
) where {P<:PaddableLayer,AoN<:Union{AbstractArray,Nothing}}
ndim = Ai
if Ai == nothing
Ai = cLayer.prevLayer.A
end
if cLayer.padding == :same
Ai = padding(Ai, paddingSize(cLayer, Ai)...)
elseif cLayer.padding == :valid
Ai = Ai
end
return Ai
end #function padding(cLayer::P) where {P <: PaddableLayer}
"""
function padding(Ai::AbstractArray{T,4},
p_H::Integer,
p_W::Integer=-1) where {T}
pad zeros to the Array Ai with amount of p values
inputs:
Ai := Array of type T and dimension N
p := integer determinde the amount of zeros padding
i.e.
if Ai is a 3-dimensional array the padding will be for the first
dimension
if Ai is a 4-dimensional array the padding will be for the first 2
dimensions
if Ai is a 5-dimensional array the padding will be for the first 3
dimensions
output:
PaddinView array where it contains the padded values and the original
data without copying it
"""
function padding(
Ai::AbstractArray{T,4},
p_H_hi::Integer,
p_H_lo::Integer,
p_W_hi::Integer,
p_W_lo::Integer,
) where {T}
n_H, n_W, c, m = size(Ai)
return PaddedView(
0,
Ai,
(p_H_hi + p_H_lo + n_H, p_W_hi + p_W_lo + n_W, c, m),
(1 + p_H_hi, 1 + p_W_hi, 1, 1),
)
end #function padding(Ai::Array{T,4}
function padding(
Ai::AbstractArray{T,3},
p_H_hi::Integer,
p_H_lo::Integer,
) where {T}
n_H, c, m = size(Ai)
return PaddedView(0, Ai, (p_H_hi + p_H_lo + n_H, c, m), (1 + p_H_hi, 1, 1))
end #function padding(Ai::Array{T,3}
function padding(
Ai::AbstractArray{T,5},
p_H_hi::Integer,
p_H_lo::Integer,
p_W_hi::Integer,
p_W_lo::Integer,
p_D_hi::Integer,
p_D_lo::Integer,
) where {T}
n_H, n_W, n_D, c, m = size(Ai)
return PaddedView(
0,
Ai,
(
p_H_hi + p_H_lo + n_H,
p_W_hi + p_W_lo + n_W,
p_D_hi + p_D_lo + n_D,
c,
m,
),
(1 + p_H_hi, 1 + p_W_hi, 1 + p_D_hi, 1, 1),
)
end #function padding(Ai::Array{T,5}
export padding
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 9415 | #Naive convolution method using for loops with some parallel using
#@simd and @inbounds
function convolve(cLayer::Conv1D, Ai::AbstractArray{T1,3}) where {T1}
Aip = padding(cLayer, Ai)
f_H = cLayer.f
s_H = cLayer.s
lastDim = ndims(Ai)
paddedS = paddedSize(cLayer, Ai)[1:end-2]
s = cLayer.s
f = cLayer.f
outputS = outDims(cLayer, Ai)[1:end-2]
ci, m = cLayer.inputS[end-1:end]
co = cLayer.channels
Z = zeros(eltype(Ai), outputS..., co, m)
n_H, c, m = size(Z)
W = cLayer.W
B = cLayer.B
# @simd
for mi = 1:m
# @simd for
Threads.@threads for ci = 1:c
# @simd
for hi = 1:n_H
h_start = hi * s_H - (s_H == 1 ? 0 : s_H - 1)
h_end = hi * s_H - (s_H == 1 ? 0 : s_H - 1) + f_H - 1
@inbounds ai = view(Aip,h_start:h_end, :, mi)
@inbounds Z[hi, ci, mi] = W[:, :, ci] ⋅ ai + B[1,1,ci]
# ai = nothing
# Base.GC.gc()
end #for mi=1:m, hi=1:n_H
end #for ci=1:c
end #for mi=1:m
# @inbounds Z .+= B[:, 1, :]
actFun = cLayer.actFun
Ao = eval(:($actFun($Z)))
# Base.GC.gc()
return Dict(:Z => Z, :A => Ao)
end #function convolve(cLayer::Conv1D
function convolve(cLayer::Conv2D, Ai::AbstractArray{T1,4}) where {T1}
Aip = padding(cLayer, Ai)
f_H, f_W = cLayer.f
s_H, s_W = cLayer.s
lastDim = ndims(Ai)
cLayer.inputS = size(Ai)
paddedS = paddedSize(cLayer, Ai)[1:end-2]
s = cLayer.s
f = cLayer.f
outputS = outDims(cLayer, Ai)[1:end-2]
ci, m = cLayer.inputS[end-1:end]
co = cLayer.channels
Z = zeros(eltype(Ai), outputS..., co, m)
n_H, n_W, c, m = size(Z)
W = cLayer.W
B = cLayer.B
# @simd
for mi = 1:m
# @simd
Threads.@threads for ci = 1:c
# @simd for
# wi = 1:n_W
for wi = 1:n_W, hi = 1:n_H
# @simd
# for hi = 1:n_H
h_start = hi * s_H - (s_H == 1 ? 0 : s_H - 1)
h_end = hi * s_H - (s_H == 1 ? 0 : s_H - 1) + f_H - 1
w_start = wi * s_W - (s_W == 1 ? 0 : s_W - 1)
w_end = wi * s_W - (s_W == 1 ? 0 : s_W - 1) + f_W - 1
@inbounds ai = view(Aip,h_start:h_end, w_start:w_end, :, mi)
# @inbounds ai = Aip[h_start:h_end, w_start:w_end, :, mi]
@inbounds Z[hi, wi, ci, mi] = W[:, :, :, ci] ⋅ ai + B[1,1,1,ci]
# end #for hi=1:n_H
end #for wi=1:n_W, hi=1:n_H
end #for ci=1:c
end #for mi=1:m
# @inbounds Z .+= B[:, :, 1, :]
actFun = cLayer.actFun
Ao = eval(:($actFun($Z)))
# Base.GC.gc()
return Dict(:Z => Z, :A => Ao)
end #function convolve(cLayer::Conv2D
function convolve(cLayer::Conv3D, Ai::AbstractArray{T1,5}) where {T1}
Aip = padding(cLayer, Ai)
f_H, f_W, f_D = cLayer.f
s_H, s_W, s_D = cLayer.s
lastDim = ndims(Ai)
paddedS = paddedSize(cLayer, Ai)[1:end-2]
s = cLayer.s
f = cLayer.f
outputS = outDims(cLayer, Ai)[1:end-2]
ci, m = cLayer.inputS[end-1:end]
co = cLayer.channels
Z = zeros(eltype(Ai), outputS..., co, m)
n_H, n_W, n_D, c, m = size(Z)
W = cLayer.W
B = cLayer.B
# @simd
for mi = 1:m
# @simd for
Threads.@threads for ci = 1:c
# @simd for
# @simd for
for di = 1:n_D, wi = 1:n_W, hi = 1:n_H
h_start = hi * s_H - (s_H == 1 ? 0 : s_H - 1)
h_end = hi * s_H - (s_H == 1 ? 0 : s_H - 1) + f_H - 1
w_start = wi * s_W - (s_W == 1 ? 0 : s_W - 1)
w_end = wi * s_W - (s_W == 1 ? 0 : s_W - 1) + f_W - 1
d_start = di * s_D - (s_D == 1 ? 0 : s_D - 1)
d_end = di * s_D - (s_D == 1 ? 0 : s_D - 1) + f_D - 1
@inbounds ai = view(Aip,
h_start:h_end,
w_start:w_end,
d_start:d_end,
:,
mi,
)
@inbounds Z[hi, wi, di, ci, mi] =
W[:, :, :, :, ci] ⋅ ai + B[1,1,1,1,ci]
# ai = nothing
# Base.GC.gc()
end #for hi=1:n_H
# end #for wi=1:n_W
# end #for di=1:n_D
end #for ci=1:c
end #for mi=1:m
# @inbounds Z .+= B[:, :, :, 1, :]
actFun = cLayer.actFun
Ao = eval(:($actFun($Z)))
# Base.GC.gc()
return Dict(:Z => Z, :A => Ao)
end #function convolve(cLayer::Conv3D
export convolve
### dconvolve
#Naive convolution method using for loops with some parallel using
#@simd and @inbounds
function dconvolve!(
cLayer::Conv1D,
Ai::AbstractArray{T1,3},
dAi::AbstractArray{T2,3},
dZ::AbstractArray{T3,3},
) where {T1,T2,T3}
Aip = padding(cLayer, Ai)
padS = paddingSize(cLayer, Ai)
dAip = zeros(promote_type(eltype(dZ),eltype(Ai)), size(Aip))
f_H = cLayer.f
s_H = cLayer.s
lastDim = ndims(Ai)
n_H, c, m = size(dZ)
W = cLayer.W
cLayer.dW = similar(W)
cLayer.dW .= 0
B = cLayer.B
cLayer.dB = similar(B)
cLayer.dB .= 0
# @simd
for mi = 1:m
# @simd
Threads.@threads for ci = 1:c
for hi = 1:n_H
h_start = hi * s_H - (s_H == 1 ? 0 : s_H - 1)
h_end = hi * s_H - (s_H == 1 ? 0 : s_H - 1) + f_H - 1
@inbounds ai = view(Aip, h_start:h_end, :, mi)
@inbounds dAip[h_start:h_end, :, mi] .+=
dZ[hi, ci, mi] .* W[:, :, ci]
@inbounds cLayer.dW[:, :, ci] .+= (ai .* dZ[hi, ci, mi])
@inbounds cLayer.dB[:, :, ci] .+= dZ[hi, ci, mi]
end #for
end #for ci=1:c
end #for mi=1:m
dAi .= dAip[1+padS[1]:end-padS[2], :, :]
return dAi
end #function dconvolve(cLayer::Conv1D
function dconvolve!(
cLayer::Conv2D,
Ai::AbstractArray{T1,4},
dAi::AbstractArray{T2,4},
dZ::AbstractArray{T3,4},
) where {T1,T2,T3}
Aip = padding(cLayer, Ai)
padS = paddingSize(cLayer, Ai)
dAip = zeros(promote_type(eltype(dZ),eltype(Ai)), size(Aip))
f_H, f_W = cLayer.f
s_H, s_W = cLayer.s
lastDim = ndims(Ai)
n_H, n_W, c, m = size(dZ)
W = cLayer.W
cLayer.dW = similar(W)
cLayer.dW .= 0
B = cLayer.B
cLayer.dB = similar(B)
cLayer.dB .= 0
# @simd
for mi = 1:m
# @simd
Threads.@threads for ci = 1:c
for wi = 1:n_W, hi = 1:n_H
h_start = hi * s_H - (s_H == 1 ? 0 : s_H - 1)
h_end = hi * s_H - (s_H == 1 ? 0 : s_H - 1) + f_H - 1
w_start = wi * s_W - (s_W == 1 ? 0 : s_W - 1)
w_end = wi * s_W - (s_W == 1 ? 0 : s_W - 1) + f_W - 1
@inbounds ai = view(Aip, h_start:h_end, w_start:w_end, :, mi)
@inbounds dAip[h_start:h_end, w_start:w_end, :, mi] .+=
dZ[hi, wi, ci, mi] .* W[:, :, :, ci]
@inbounds cLayer.dW[:, :, :, ci] .+= (ai .* dZ[hi, wi, ci, mi])
@inbounds cLayer.dB[:, :, :, ci] .+= dZ[hi, wi, ci, mi]
end #for
end #for ci=1:c
end #for mi=1:m
dAi .= dAip[1+padS[1]:end-padS[2], 1+padS[3]:end-padS[4], :, :]
return dAi
end #function dconvolve(cLayer::Conv2D
function dconvolve!(
cLayer::Conv3D,
Ai::AbstractArray{T1,5},
dAi::AbstractArray{T2,5},
dZ::AbstractArray{T3,5},
) where {T1,T2,T3}
Aip = padding(cLayer, Ai)
padS = paddingSize(cLayer, Ai)
dAip = zeros(promote_type(eltype(dZ),eltype(Ai)), size(Aip))
f_H, f_W, f_D = cLayer.f
s_H, s_W, s_D = cLayer.s
lastDim = ndims(Ai)
n_H, n_W, n_D, c, m = size(dZ)
W = cLayer.W
cLayer.dW = similar(W)
cLayer.dW .= 0
B = cLayer.B
cLayer.dB = similar(B)
cLayer.dB .= 0
# @simd
for mi = 1:m
# @simd
Threads.@threads for ci = 1:c
for di = 1:n_D, wi = 1:n_W, hi = 1:n_H
h_start = hi * s_H - (s_H == 1 ? 0 : s_H - 1)
h_end = hi * s_H - (s_H == 1 ? 0 : s_H - 1) + f_H - 1
w_start = wi * s_W - (s_W == 1 ? 0 : s_W - 1)
w_end = wi * s_W - (s_W == 1 ? 0 : s_W - 1) + f_W - 1
d_start = di * s_D - (s_D == 1 ? 0 : s_D - 1)
d_end = di * s_D - (s_D == 1 ? 0 : s_D - 1) + f_D - 1
@inbounds ai =
view(Aip, h_start:h_end, w_start:w_end, d_start:d_end, :, mi)
@inbounds dAip[
h_start:h_end,
w_start:w_end,
d_start:d_end,
:,
mi,
] .+= dZ[hi, wi, di, ci, mi] .* W[:, :, :, :, ci]
@inbounds cLayer.dW[:, :, :, :, ci] .+=
(ai .* dZ[hi, wi, ci, mi])
@inbounds cLayer.dB[:, :, :, :, ci] .+= dZ[hi, wi, di, ci, mi]
end #for
end #for ci=1:c
end #for mi=1:m
dAi .= dAip[1+padS[1]:end-padS[2],
1+padS[3]:end-padS[4],
1+padS[5]:end-padS[6],
:,
:,
]
return nothing
end #function dconvolve(cLayer::Conv3D
export dconvolve!
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 5129 | using Statistics
function fastPooling!(
cLayer::OneD,
Ai::AbstractArray{T,3},
Ao::AbstractArray{T,3},
) where {OneD<:Union{MaxPool1D,AveragePool1D},T}
Aip = padding(cLayer, Ai)
n_Hi, c, m = size(Aip)
s_H = cLayer.s
f_H = cLayer.f
n_H = (n_Hi - f_H) ÷ s_H + 1
if cLayer isa MaxPoolLayer
pool = maximum
else
pool = mean
end
Ao .= reshape(
pool(reshape(Aip, f_H, n_H, c, m), dims = 1),
n_H,
c,
m,
)
return nothing
end #function fastPooling!(cLayer::OneD
function fastPooling!(
cLayer::TwoD,
Ai::AbstractArray{T,4},
Ao::AbstractArray{T,4},
) where {TwoD<:Union{MaxPool2D,AveragePool2D},T}
Aip = padding(cLayer, Ai)
n_Hi, n_Wi, c, m = size(Aip)
s_H, s_W = cLayer.s
f_H, f_W = cLayer.f
n_H = (n_Hi - f_H) ÷ s_H + 1
n_W = (n_Wi - f_W) ÷ s_W + 1
if cLayer isa MaxPoolLayer
pool = maximum
else
pool = mean
end
Ao .= reshape(
pool(
permutedims(
reshape(Aip, f_H, n_H, f_W, n_W, c, m),
[1, 3, 2, 4, 5, 6],
),
dims = 1:2,
),
n_H,
n_W,
c,
m,
)
return nothing
end #function fastPooling!(cLayer::TwoD,
function fastPooling!(
cLayer::ThreeD,
Ai::AbstractArray{T,5},
Ao::AbstractArray{T,5},
) where {ThreeD<:Union{MaxPool3D,AveragePool3D},T}
Aip = padding(cLayer, Ai)
n_Hi, n_Wi, n_Di, c, m = size(Aip)
s_H, s_W, s_D = cLayer.s
f_H, f_W, f_D = cLayer.f
n_H = (n_Hi - f_H) ÷ s_H + 1
n_W = (n_Wi - f_W) ÷ s_W + 1
n_D = (n_Di - f_D) ÷ s_D + 1
if cLayer isa MaxPoolLayer
pool = maximum
else
pool = mean
end
Ao .= reshape(
pool(
permutedims(
reshape(Aip, f_H, n_H, f_W, n_W, f_D, n_D, c, m),
[1, 3, 5, 2, 4, 6, 7, 8],
),
dims = 1:3,
),
n_H,
n_W,
n_D,
c,
m,
)
return nothing
end #function fastPooling!(cLayer::ThreeD,
export fastPooling!
### dfastPooling
function dfastPooling!(
cLayer::OneD,
Ai::AbstractArray{T,3},
dAi::AbstractArray{T,3},
Ao::AbstractArray{T,3},
dAo::AbstractArray{T,3},
) where {OneD<:Union{MaxPool1D,AveragePool1D},T}
padS = paddingSize(cLayer, Ai)
Aip = padding(cLayer, Ai)
n_Hi, c, m = size(Aip)
s_H = cLayer.s
f_H = cLayer.f
n_H = (n_Hi - f_H) ÷ s_H + 1
Aipr = reshape(Aip, f_H, n_H, c, m)
if cLayer isa MaxPoolLayer
mask = Aipr .== reshape(Ao, 1, n_H, c, m)
else
mask = similar(Aipr) .= 1 / prod((cLayer.f))
end #if cLayer isa MaxPoolLayer
dAi .= reshape(reshape(dAo, 1, n_H, c, m) .* mask, n_Hi, c, m)[1+padS[1]:end-padS[2], :, :]
mask = nothing
return nothing
end #function dfastPooling!(cLayer::OneD
function dfastPooling!(
cLayer::TwoD,
Ai::AbstractArray{T,4},
dAi::AbstractArray{T,4},
Ao::AbstractArray{T,4},
dAo::AbstractArray{T,4},
) where {TwoD<:Union{MaxPool2D,AveragePool2D},T}
padS = paddingSize(cLayer, Ai)
Aip = padding(cLayer, Ai)
n_Hi, n_Wi, c, m = size(Aip)
s_H, s_W = cLayer.s
f_H, f_W = cLayer.f
n_H = (n_Hi - f_H) ÷ s_H + 1
n_W = (n_Wi - f_W) ÷ s_W + 1
Aipr =
permutedims(reshape(Aip, f_H, n_H, f_W, n_W, c, m), [1, 3, 2, 4, 5, 6])
if cLayer isa MaxPoolLayer
mask = Aipr .== reshape(Ao, 1, 1, n_H, n_W, c, m)
else
mask = similar(Aipr) .= 1 / prod((cLayer.f))
end
dAi .= reshape(
permutedims(
reshape(dAo, 1, 1, n_H, n_W, c, m) .* mask,
[1, 3, 2, 4, 5, 6],
),
n_Hi,
n_Wi,
c,
m,
)[1+padS[1]:end-padS[2], 1+padS[3]:end-padS[4], :, :]
mask = nothing
return nothing
end #function dfastPooling!(cLayer::TwoD,
function dfastPooling!(
cLayer::ThreeD,
Ai::AbstractArray{T,5},
dAi::AbstractArray{T,5},
Ao::AbstractArray{T,5},
dAo::AbstractArray{T,5},
) where {ThreeD<:Union{MaxPool3D,AveragePool3D},T}
padS = paddingSize(cLayer, Ai)
Aip = padding(cLayer, Ai)
n_Hi, n_Wi, n_Di, c, m = size(Aip)
s_H, s_W, s_D = cLayer.s
f_H, f_W, f_D = cLayer.f
n_H = (n_Hi - f_H) ÷ s_H + 1
n_W = (n_Wi - f_W) ÷ s_W + 1
n_D = (n_Di - f_D) ÷ s_D + 1
Aipr = permutedims(
reshape(Aip, f_H, n_H, f_W, n_W, f_D, n_D, c, m),
[1, 3, 5, 2, 4, 6, 7, 8],
)
if cLayer isa MaxPoolLayer
mask = Aipr .== reshape(Ao, 1, 1, 1, n_H, n_W, n_D, c, m)
else
mask = similar(Aipr) .= 1 / prod((cLayer.f))
end
dAi .= reshape(
permutedims(
reshape(dAo, 1, 1, 1, n_H, n_W, n_D, c, m) .* mask,
[1, 4, 2, 5, 3, 6, 7, 8],
),
n_Hi,
n_Wi,
n_Di,
c,
m,
)[1+padS[1]:end-padS[2],
1+padS[3]:end-padS[4],
1+padS[5]:end-padS[6],
:,
:,
]
mask = nothing
return nothing
end #function dfastPooling!(cLayer::ThreeD,
export dfastPooling!
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 2991 |
function img2colConvolve(cLayer::CL, Ai::AbstractArray{T,N}) where {T,N, CL <: ConvLayer}
Aip = padding(cLayer, Ai)
axBT = axes(cLayer.B)
axB = axBT[1:end-2]
axBend = axBT[end]
Z = col2img(cLayer.K * img2col(Aip), cLayer.outputS) .+
view(cLayer.B, axB..., 1, axBend)
actFun = cLayer.actFun
Ao = eval(:($actFun($Z)))
return Dict(:Z => Z, :A => Ao)
end #function img2colConvolve(cLayer::CL
export img2colConvolve
### dimg2colConvolve!
export dimg2colConvolve!
function dimg2colConvolve!(
cLayer::Conv1D,
Ai::AbstractArray{T1,3},
dAi::AbstractArray{T2,3},
dZ::AbstractArray{T3,3},
) where {T1,T2,T3}
padS = paddingSize(cLayer, Ai)
Aip = padding(cLayer, Ai)
Aipv = img2col(Aip)
dZv = img2col(dZ)
n_Hi, c, m = size(Aip)
s_H = cLayer.s
f_H = cLayer.f
n_H = (n_Hi - f_H) ÷ s_H + 1
dAi .= col2img(cLayer.K' * dZv, (n_Hi,c,m))[1+padS[1]:end-padS[2], :, :]
cLayer.dK = dZv * Aipv'
cLayer.dB = sum(permutedims(dZ, [1, 3, 2]), dims = 1:2)
return nothing
end # function dimg2colConvolve!(
# cLayer::Conv1D,
# Ai::AbstractArray{T,3},
# dA::AbstractArray{T,3},
# dZ::AbstractArray{T,3},
# ) where {T}
function dimg2colConvolve!(
cLayer::Conv2D,
Ai::AbstractArray{T1,4},
dAi::AbstractArray{T2,4},
dZ::AbstractArray{T3,4},
) where {T1,T2,T3}
padS = paddingSize(cLayer, Ai)
Aip = padding(cLayer, Ai)
Aipv = img2col(Aip)
dZv = img2col(dZ)
n_Hi, n_Wi, c, m = size(Aip)
s_H, s_W = cLayer.s
f_H, f_W = cLayer.f
n_H = (n_Hi - f_H) ÷ s_H + 1
n_W = (n_Wi - f_W) ÷ s_W + 1
dAi .= col2img(cLayer.K' * dZv, (n_Hi,n_Wi,c,m))[1+padS[1]:end-padS[2], 1+padS[3]:end-padS[4], :, :]
cLayer.dK = dZv * Aipv'
cLayer.dB = sum(permutedims(dZ, [1, 2, 4, 3]), dims = 1:3)
return nothing
end # function dimg2colConvolve!(
# cLayer::Conv2D,
# Ai::AbstractArray{T,4},
# dA::AbstractArray{T,4},
# dZ::AbstractArray{T,4},
# ) where {T}
function dimg2colConvolve!(
cLayer::Conv3D,
Ai::AbstractArray{T1,5},
dAi::AbstractArray{T2,5},
dZ::AbstractArray{T3,5},
) where {T1,T2,T3}
padS = paddingSize(cLayer, Ai)
Aip = padding(cLayer, Ai)
Aipv = img2col(Aip)
dZv = img2col(dZ)
n_Hi, n_Wi, n_Di, c, m = size(Aip)
s_H, s_W, s_D = cLayer.s
f_H, f_W, f_D = cLayer.f
n_H = (n_Hi - f_H) ÷ s_H + 1
n_W = (n_Wi - f_W) ÷ s_W + 1
n_D = (n_Di - f_D) ÷ s_D + 1
dAi .= col2img(cLayer.K' * dZv, (n_Hi, n_Wi, n_Di, c, m))[1+padS[1]:end-padS[2],
1+padS[3]:end-padS[4],
1+padS[5]:end-padS[6],
:,
:,
]
cLayer.dK = dZv * Aipv'
cLayer.dB = sum(permutedims(dZ, [1, 2, 3, 5, 4]), dims = 1:4)
return nothing
end # function dimg2colConvolve!(
# cLayer::Conv3D,
# Ai::AbstractArray{T,5},
# dA::AbstractArray{T,5},
# dZ::AbstractArray{T,5},
# ) where {T}
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 6632 |
### convlayers
@doc raw"""
function layerBackProp(
cLayer::ConvLayer,
model::Model,
actFun::SoS,
Ao::AbstractArray,
labels::AbstractArray,
) where {SoS<:Union{Type{softmax},Type{σ}}}
Derive the loss function to the input of the activation function when activation is either `softmax` or `σ`
# Return
- `dZ::AbstractArray` := the derivative of the loss function to the input of the activation function
"""
function layerBackProp(
cLayer::ConvLayer,
model::Model,
actFun::SoS,
Ao::AbstractArray,
labels::AbstractArray,
) where {SoS<:Union{Type{softmax},Type{σ}}}
lossFun = model.lossFun
dlossFun = Symbol("d", lossFun)
Y = labels
dZ = eval(:($dlossFun($Ao, $Y)))
return dZ
end #softmax or σ layerBackProp
@doc raw"""
function layerBackProp(
cLayer::ConvLayer,
model::Model,
FCache::Dict{Layer, Dict{Symbol, AbstractArray}},
BCache::Dict{Layer, Dict{Symbol, AbstractArray}},
Ai::AbstractArray = Array{Any,1}(undef,0),
Ao::AbstractArray = Array{Any,1}(undef,0),
dAo::AbstractArray = Array{Any,1}(undef,0);
labels::AbstractArray = Array{Any,1}(undef,0),
kwargs...
)
Performs the layer back propagation for a `ConvLayer`
# Arguments
- `cLayer::ConvLayer`
- `model::Model`
- `FCache` := the cache of the forward propagation step
- `BCache` := the cache of so far done back propagation
- for test purpose
Ai
Ao
dAo
- `labels` := when `cLayer` is an output `Layer`
# Return
- `Dict(:dA => dAi)`
"""
function layerBackProp(
cLayer::CL,
model::Model,
FCache::Dict{Layer, Dict{Symbol, AbstractArray}},
BCache::Dict{Layer, Dict{Symbol, AbstractArray}},
Ai::AbstractArray = Array{Any,1}(undef,0),
Ao::AbstractArray = Array{Any,1}(undef,0),
dAo::AbstractArray = Array{Any,1}(undef,0);
labels::AbstractArray = Array{Any,1}(undef,0),
kwargs...
) where {CL <: ConvLayer}
kwargs = Dict{Symbol, Any}(kwargs...)
NNlib = getindex(kwargs, :NNlib; default=true)
img2col = getindex(kwargs, :img2col; default=true)
if length(Ai) == 0
Ai = FCache[cLayer.prevLayer][:A]
end
if length(Ao) == 0
Ao = FCache[cLayer][:A]
end
m = size(Ao)[end]
regulization, λ = model.regulization, model.λ
actFun = cLayer.actFun
dZ = []
if cLayer.actFun == model.outLayer.actFun
dZ = layerBackProp(cLayer, model, eval(:($actFun)), Ao, labels)
elseif length(dAo) != 0
dActFun = Symbol("d", cLayer.actFun)
Z = FCache[cLayer][:Z]
dZ = dAo .* eval(:($dActFun($Z)))
elseif all(
i -> (i.backCount == cLayer.nextLayers[1].backCount),
cLayer.nextLayers,
)
dAo = []
for nextLayer in cLayer.nextLayers
try
dAo .+= getLayerSlice(cLayer, nextLayer, BCache)
catch e
dAo = getLayerSlice(cLayer, nextLayer, BCache) #need to initialize dA
end #try/catch
end #for
dActFun = Symbol("d", cLayer.actFun)
Z = FCache[cLayer][:Z]
dZ = dAo .* eval(:($dActFun($Z)))
else #in case not every next layer done backprop
return Dict(:dA => Array{Any,1}(undef,0))
end #if all(i->(i.backCount==cLayer.nextLayers[1].backCount), cLayer.nextLayers)
dAi = zeros(promote_type(eltype(Ai),eltype(dZ)), size(Ai))
if NNlib
dNNConv!(cLayer, Ai, dAi, dZ)
elseif img2col
dimg2colConvolve!(cLayer, Ai, dAi, dZ)
else
dconvolve!(cLayer, Ai, dAi, dZ)
end #if NNlib
cLayer.backCount += 1
return Dict(:dA => dAi)
end #function layerBackProp(cLayer::Input
### Pooling Layers
#import only the needed parts not to have conflict
import NNlib.∇maxpool, NNlib.∇meanpool, NNlib.∇maxpool!, NNlib.∇meanpool!, NNlib.PoolDims
@doc raw"""
layerBackProp(
cLayer::PoolLayer,
model::Model,
FCache::Dict{Layer, Dict{Symbol, AbstractArray}},
BCache::Dict{Layer, Dict{Symbol, AbstractArray}},
Ai::AbstractArray = Array{Any,1}(undef,0),
Ao::AbstractArray = Array{Any,1}(undef,0),
dAo::AbstractArray = Array{Any,1}(undef,0);
labels::AbstractArray = Array{Any,1}(undef,0),
kwargs...
)
Performs the layer back propagation for a `PoolLayer`
# Arguments
- `cLayer::ConvLayer`
- `model::Model`
- `FCache` := the cache of the forward propagation step
- `BCache` := the cache of so far done back propagation
- for test purpose
Ai
Ao
dAo
- `labels` := when `cLayer` is an output `Layer`
# Return
- `Dict(:dA => dAi)`
"""
function layerBackProp(
cLayer::PL,
model::Model,
FCache::Dict{Layer, Dict{Symbol, AbstractArray}},
BCache::Dict{Layer, Dict{Symbol, AbstractArray}},
Ai::AbstractArray = Array{Any,1}(undef,0),
Ao::AbstractArray = Array{Any,1}(undef,0),
dAo::AbstractArray = Array{Any,1}(undef,0);
labels::AbstractArray = Array{Any,1}(undef,0),
kwargs...
) where {PL <: PoolLayer}
kwargs = Dict{Symbol, Any}(kwargs...)
fastPool = getindex(kwargs, :fastPool; default=true)
NNlib = getindex(kwargs, :NNlib; default=true)
if length(Ai) == 0
Ai = FCache[cLayer.prevLayer][:A]
end
if length(Ao) == 0
Ao = FCache[cLayer][:A]
end
padS = paddingSize(cLayer, Ai)
if length(dAo) == 0
if all(
i -> (i.backCount == cLayer.nextLayers[1].backCount),
cLayer.nextLayers,
)
dAo = []
for nextLayer in cLayer.nextLayers
try
dAo .+= getLayerSlice(cLayer, nextLayer, BCache)
catch e
dAo = getLayerSlice(cLayer, nextLayer, BCache) #need to initialize dA
end #try/catch
end #for
else
return Dict(:dA => Array{Any,1}(undef,0))
end #if all(i->(i.backCount==cLayer.nextLayers[1].backCount), cLayer.nextLayers)
end #if dA==nothing
dAi = zeros(promote_type(eltype(Ai), eltype(dAo)), size(Ai))
if NNlib
pooldims = PoolDims(Ai, cLayer.f, stride = cLayer.s, padding = padS)
if cLayer isa MaxPoolLayer
∇maxpool!(dAi, dAo, Ao, Ai, pooldims)
elseif cLayer isa AveragePoolLayer
∇meanpool!(dAi, dAo, Ao, Ai, pooldims)
end #if cLayer isa MaxPoolLayer
elseif cLayer.s == cLayer.f && fastPool
dfastPooling!(cLayer, Ai, dAi, Ao, dAo)
else
dpooling!(cLayer, Ai, dAi, Ao, dAo)
end #if NNlib
cLayer.backCount += 1
return Dict(:dA => dAi)
end #unction layerBackProp(cLayer::PL,
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 3816 | include("parallelNNConv.jl")
include("parallelConvolve.jl")
include("parallelImg2colConvolve.jl")
###convolution layers forprop
@doc raw"""
function layerForProp(
cLayer::ConvLayer,
Ai::AbstractArray = Array{Any,1}(undef,0);
FCache::Dict{Layer,Dict{Symbol, AbstractArray}},
kwargs...
)
Perform the layer forward propagation for a `ConvLayer`
# Arguments
- `cLayer::ConvLayer`
- `Ai` := optional activation of the previous layer
- `FCache` := a `Dict` holds the outputs of `layerForProp` of the previous `Layer`(s)
# Returns
- `Dict(:Z => Z, :A => Ao)`
"""
function layerForProp(
cLayer::CL,
Ai::AbstractArray = Array{Any,1}(undef,0);
FCache::Dict{Layer,Dict{Symbol, AbstractArray}},
kwargs...
) where {CL <: ConvLayer}
kwargs = Dict{Symbol, Any}(kwargs...)
NNlib = getindex(kwargs, :NNlib; default=true)
img2col = getindex(kwargs, :img2col; default=false)
if length(Ai) == 0
Ai = FCache[cLayer.prevLayer][:A]
end
cLayer.inputS = size(Ai)
paddedS = paddedSize(cLayer, Ai)[1:end-2]
s = cLayer.s
f = cLayer.f
outputS = outDims(cLayer, Ai)[1:end-2]
ci, m = cLayer.inputS[end-1:end]
co = cLayer.channels
if NNlib
D = NNConv(cLayer, Ai)
elseif img2col
## in case input different size than previous time
if (prod((outputS..., co)), prod((paddedS..., ci))) != size(cLayer.K)
cLayer.K = unroll(cLayer, (paddedS..., ci, m))
end
D = img2colConvolve(cLayer, Ai)
else
D = convolve(cLayer, Ai)
end
cLayer.outputS = size(D[:A])
# Ai = nothing
cLayer.forwCount += 1
# Base.GC.gc()
return D
end #function layerForProp(cLayer::Conv1D)
### Pooling Layers
include("parallelFastPooling.jl")
include("parallelPooling.jl")
#import only the needed parts not to have conflict
import NNlib.maxpool, NNlib.meanpool, NNlib.maxpool!, NNlib.meanpool!, NNlib.PoolDims
@doc raw"""
layerForProp(
cLayer::PoolLayer},
Ai::AbstractArray = Array{Any,1}(undef,0);
FCache::Dict{Layer,Dict{Symbol, AbstractArray}},
kwargs...
)
Perform the layer forward propagation for a `PoolLayer`
# Arguments
- `cLayer::PoolLayer`
- `Ai` := optional activation of the previous layer
- `FCache` := a `Dict` holds the outputs of `layerForProp` of the previous `Layer`(s)
# Returns
- `Dict(:A => Ao)`
"""
function layerForProp(
cLayer::PL,
Ai::AbstractArray = Array{Any,1}(undef,0);
FCache::Dict{Layer,Dict{Symbol, AbstractArray}},
kwargs...
) where {PL <: PoolLayer}
kwargs = Dict{Symbol, Any}(kwargs...)
fastPool = getindex(kwargs, :fastPool; default=true)
NNlib = getindex(kwargs, :NNlib; default=true)
if length(Ai) == 0
Ai = FCache[cLayer.prevLayer][:A]
end
cLayer.inputS = size(Ai)
paddedS = paddedSize(cLayer, Ai)[1:end-2]
s = cLayer.s
f = cLayer.f
outputS = outDims(cLayer, Ai)[1:end-2]
ci, m = cLayer.inputS[end-1:end]
co = cLayer.channels
cLayer.inputS = size(Ai)
padS = paddingSize(cLayer, Ai)
Ao = zeros(eltype(Ai), outputS..., co, m)
if NNlib
pooldims = PoolDims(Ai, f, stride = s, padding = padS)
if cLayer isa MaxPoolLayer
maxpool!(Ao, Ai, pooldims)
elseif cLayer isa AveragePoolLayer
meanpool!(Ao, Ai, pooldims)
end #if cLayer isa MaxPoolLayer
elseif s == f && fastPool #to use the built-in reshape and maximum and mean
fastPooling!(cLayer, Ai, Ao)
else
pooling!(cLayer, Ai, Ao)
end #if NNlibConv
cLayer.outputS = size(Ao)
# Ai = nothing
cLayer.forwCount += 1
# Base.GC.gc()
return Dict(:A => Ao)
end #unction layerForProp(cLayer::OneD) where {OneD <: Union{MaxPool1D, AveragePool1D}}
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 2310 | #import only the needed parts not to have conflict
import NNlib.conv, NNlib.conv!
### NNConv
@doc raw"""
NNConv(cLayer::CL, Ai::AbstractArray{T,N}) where {T,N, CL <: ConvLayer}
Perform the forward propagation for `cLayer::ConvLayer` using fast implementation of `NNlib`
# Return
- `Dict(:Z => Z, :A => A)`
"""
function NNConv(cLayer::CL, Ai::AbstractArray{T,N}) where {T,N, CL <: ConvLayer}
padS = paddingSize(cLayer, Ai)
# axW = axes(cLayer.W)[1:end-2]
# raxW = reverse.(axW)
# Z = conv(Ai, cLayer.W[raxW..., :, :], stride = cLayer.s, pad = padS)
Z = conv(Ai, cLayer.W, stride = cLayer.s, pad = padS, flipped=true)
axB = axes(cLayer.B)[1:end-2]
Z .+= cLayer.B[axB..., 1, :]
actFun = cLayer.actFun
A = eval(:($actFun($Z)))
return Dict(:Z => Z, :A => A)
end #function img2colConvolve(cLayer::CL
export NNConv
### dNNConv!
#import only the needed parts not to have conflict
import NNlib.∇conv_data!, NNlib.∇conv_filter, NNlib.DenseConvDims
@doc raw"""
function dNNConv!(
cLayer::CL,
Ai::AbstractArray{T1,N},
dAi::AbstractArray{T2,N},
dZ::AbstractArray{T3,N},
) where {T1, T2, T3, N, CL <: ConvLayer}
Performs the back propagation for `cLayer::ConvLayer` and save values to the pre-allocated `Array` `dAi` and trainable parameters `W` & `B`
# Arguments
- `cLayer::ConvLayer`
- `Ai::AbstractArray{T1,N}` := the input activation of `cLayer`
- `dAi::AbstractArray{T2,N}` := pre-allocated to hold the derivative of the activation
- `dZ::AbstractArray{T3,N}` := the derivative of the cost to the input of the activation function
# Return
`nothing`
"""
function dNNConv!(
cLayer::CL,
Ai::AbstractArray{T1,N},
dAi::AbstractArray{T2,N},
dZ::AbstractArray{T3,N},
) where {T1, T2, T3, N, CL <: ConvLayer}
W = cLayer.W
padS = paddingSize(cLayer, Ai)
convdim = DenseConvDims(Ai, W, stride = cLayer.s, padding = padS, flipkernel=true)
# axW = axes(cLayer.W)[1:end-2]
# raxW = reverse.(axW)
# dAi .= ∇conv_data(dZ, W[raxW..., :, :], convdim)
∇conv_data!(dAi, dZ, W, convdim)
cLayer.dW = ∇conv_filter(Ai, dZ, convdim)
cLayer.dB = sum(permutedims(dZ, [(1:N-2)..., N, N-1]), dims = 1:(N-1))
return nothing
end #function img2colConvolve(cLayer::Conv1D
export dNNConv!
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 15100 | using Statistics
function pooling!(
cLayer::OneD,
Ai::AbstractArray{T,3},
Ao::AbstractArray{T,3},
) where {OneD<:Union{MaxPool1D,AveragePool1D},T}
Aip = padding(cLayer, Ai)
n_Hi, c, m = size(Aip)
s_H = cLayer.s
f_H = cLayer.f
n_H = (n_Hi - f_H) ÷ s_H + 1
if cLayer isa MaxPoolLayer
pool = maximum
else
pool = mean
end #if cLayer isa MaxPoolLayer
# @simd
for mi = 1:m
# @simd for
Threads.@threads for ci = 1:c
# @simd
for hi = 1:n_H
h_start = hi * s_H - (s_H == 1 ? 0 : s_H - 1)
h_end = hi * s_H - (s_H == 1 ? 0 : s_H - 1) + f_H - 1
@inbounds ai = view(Aip,h_start:h_end, ci, mi)
@inbounds Ao[hi, ci, mi] = pool(ai)
# ai = nothing
# Base.GC.gc()
end #for
end #for ci=1:c
end #for mi=1:m, ci=1:c
return nothing
end #function pooling!(cLayer::OneD
function pooling!(
cLayer::TwoD,
Ai::AbstractArray{T,4},
Ao::AbstractArray{T,4},
) where {TwoD<:Union{MaxPool2D,AveragePool2D},T}
Aip = padding(cLayer, Ai)
n_Hi, n_Wi, c, m = size(Aip)
s_H, s_W = cLayer.s
f_H, f_W = cLayer.f
n_H = (n_Hi - f_H) ÷ s_H + 1
n_W = (n_Wi - f_W) ÷ s_W + 1
if cLayer isa MaxPoolLayer
pool = maximum
else
pool = mean
end #if cLayer isa MaxPoolLayer
# @simd
for mi = 1:m
# @simd for
Threads.@threads for ci = 1:c
# @simd for
# @simd
for wi = 1:n_W, hi = 1:n_H
h_start = hi * s_H - (s_H == 1 ? 0 : s_H - 1)
h_end = hi * s_H - (s_H == 1 ? 0 : s_H - 1) + f_H - 1
w_start = wi * s_W - (s_W == 1 ? 0 : s_W - 1)
w_end = wi * s_W - (s_W == 1 ? 0 : s_W - 1) + f_W - 1
@inbounds ai =
view(Aip, h_start:h_end, w_start:w_end, ci, mi)
@inbounds Ao[hi, wi, ci, mi] = pool(ai)
# ai = nothing
# Base.GC.gc()
end #for hi=1:n_H
# end #for wi=1:n_W
end #for ci=1:c
end #for mi=1:m,
return nothing
end #function pooling!(cLayer::TwoD,
function pooling!(
cLayer::ThreeD,
Ai::AbstractArray{T,5},
Ao::AbstractArray{T,5},
) where {ThreeD<:Union{MaxPool3D,AveragePool3D},T}
Aip = padding(cLayer, Ai)
n_Hi, n_Wi, n_Di, c, m = size(Aip)
s_H, s_W, s_D = cLayer.s
f_H, f_W, f_D = cLayer.f
n_H = (n_Hi - f_H) ÷ s_H + 1
n_W = (n_Wi - f_W) ÷ s_W + 1
n_D = (n_Di - f_D) ÷ s_D + 1
if cLayer isa MaxPoolLayer
pool = maximum
else
pool = mean
end #if cLayer isa MaxPoolLayer
# @simd
for mi = 1:m
# @simd for
Threads.@threads for ci = 1:c
# @simd for
# @simd for
# @simd
for di = 1:n_D, wi = 1:n_W, hi = 1:n_H
h_start = hi * s_H - (s_H == 1 ? 0 : s_H - 1)
h_end = hi * s_H - (s_H == 1 ? 0 : s_H - 1) + f_H - 1
w_start = wi * s_W - (s_W == 1 ? 0 : s_W - 1)
w_end = wi * s_W - (s_W == 1 ? 0 : s_W - 1) + f_W - 1
d_start = di * s_D - (s_D == 1 ? 0 : s_D - 1)
d_end = di * s_D - (s_D == 1 ? 0 : s_D - 1) + f_D - 1
@inbounds ai = view(Aip,
h_start:h_end,
w_start:w_end,
d_start:d_end,
ci,
mi,
)
@inbounds Ao[hi, wi, di, ci, mi] = pool(ai)
# ai = nothing
# Base.GC.gc()
end #for hi=1:n_H
# end #for wi=1:n_W
# end #for di=1:n_D
end #for ci=1:c
end #for mi=1:m, ci=1:c
return nothing
end #function pooling!(cLayer::ThreeD,
export pooling!
###dpooling
function dpooling!(
cLayer::OneD,
Ai::AbstractArray{T,3},
dAi::AbstractArray{T,3},
Ao::AbstractArray{T,3},
dAo::AbstractArray{T,3},
) where {OneD<:Union{MaxPool1D,AveragePool1D},T}
padS = paddingSize(cLayer, Ai)
paddedS = paddedSize(cLayer, Ai)
Aip = padding(cLayer, Ai)
dAip = zeros(eltype(Ai), paddedS)
n_Hi, c, m = paddedS
s_H = sS = cLayer.s
f_H = fS = cLayer.f
n_H = (n_Hi - f_H) ÷ s_H + 1
# if sS == fS #to use the @simd the parallele the operation
# # @simd
# for mi = 1:m,
# # @simd for
# ci = 1:c
# # @simd
# Threads.@threads for hi = 1:n_H
# h_start = hi * s_H - (s_H == 1 ? 0 : s_H - 1)
# h_end = hi * s_H - (s_H == 1 ? 0 : s_H - 1) + f_H - 1
# @inbounds ai = Aip[h_start:h_end, ci, mi]
# if cLayer isa MaxPoolLayer
# mask = (Ao[hi,ci,mi] .== ai)
# @inbounds dAip[h_start:h_end, ci, mi] .+=
# (dAo[hi, ci, mi] .* mask)
# else
# mask = similar(ai)
# mask .= 1 / prod(size(ai))
# @inbounds dAip[h_start:h_end, ci, mi] .+=
# (dAo[hi, ci, mi] .* mask)
# end #if cLayer isa MaxPoolLayer
# end #for hi=1:n_H
# # end #for ci=1:c
# end #for mi=1:m
# else
# @simd
for mi = 1:m
# @simd
Threads.@threads for ci = 1:c
for hi = 1:n_H
h_start = hi * s_H - (s_H == 1 ? 0 : s_H - 1)
h_end = hi * s_H - (s_H == 1 ? 0 : s_H - 1) + f_H - 1
@inbounds ai = Aip[h_start:h_end, ci, mi]
if cLayer isa MaxPoolLayer
mask = (Ao[hi,ci,mi] .== ai)
@inbounds dAip[h_start:h_end, ci, mi] .+=
(dAo[hi, ci, mi] .* mask)
else
mask = similar(ai)
mask .= 1 / prod(size(ai))
@inbounds dAip[h_start:h_end, ci, mi] .+=
(dAo[hi, ci, mi] .* mask)
end #if cLayer isa MaxPoolLayer
end #for hi=1:n_H
end #for ci=1:c
end #for mi=1:m
# end #if sS == fS
dAi .= dAip[1+padS[1]:end-padS[2], :, :]
return nothing
end #function dpooling!(cLayer::OneD
function dpooling!(
cLayer::TwoD,
Ai::AbstractArray{T,4},
dAi::AbstractArray{T,4},
Ao::AbstractArray{T,4},
dAo::AbstractArray{T,4},
) where {TwoD<:Union{MaxPool2D,AveragePool2D},T}
padS = paddingSize(cLayer, Ai)
paddedS = paddedSize(cLayer, Ai)
Aip = padding(cLayer, Ai)
dAip = zeros(eltype(Ai), paddedS)
n_Hi, n_Wi, c, m = paddedS
s_H, s_W = sS = cLayer.s
f_H, f_W = fS = cLayer.f
n_H = (n_Hi - f_H) ÷ s_H + 1
n_W = (n_Wi - f_W) ÷ s_W + 1
# if sS == fS
# # @simd
# for mi = 1:m,
# # @simd for
# ci = 1:c,
# # @simd for
# wi = 1:n_W
# # @simd
# Threads.@threads for hi = 1:n_H
# h_start = hi * s_H - (s_H == 1 ? 0 : s_H - 1)
# h_end = hi * s_H - (s_H == 1 ? 0 : s_H - 1) + f_H - 1
# w_start = wi * s_W - (s_W == 1 ? 0 : s_W - 1)
# w_end = wi * s_W - (s_W == 1 ? 0 : s_W - 1) + f_W - 1
# @inbounds ai = Aip[h_start:h_end, w_start:w_end, ci, mi]
# if cLayer isa MaxPoolLayer
# mask = (Ao[hi,wi,ci,mi] .== ai)
# @inbounds dAip[
# h_start:h_end,
# w_start:w_end,
# ci,
# mi,
# ] .+= (dAo[hi, wi, ci, mi] .* mask)
# else
# mask = similar(ai)
# mask .= 1 / prod(size(ai))
# @inbounds dAip[
# h_start:h_end,
# w_start:w_end,
# ci,
# mi,
# ] .+= (dAo[hi, wi, ci, mi] .* mask)
# end #if cLayer isa MaxPoolLayer
# end #for hi=1:n_H
# # end #for wi=1:n_W
# # end #for ci=1:c
# end #for mi=1:m
# else
# @simd
for mi = 1:m
# @simd
Threads.@threads for ci = 1:c
for wi = 1:n_W, hi = 1:n_H
h_start = hi * s_H - (s_H == 1 ? 0 : s_H - 1)
h_end = hi * s_H - (s_H == 1 ? 0 : s_H - 1) + f_H - 1
w_start = wi * s_W - (s_W == 1 ? 0 : s_W - 1)
w_end = wi * s_W - (s_W == 1 ? 0 : s_W - 1) + f_W - 1
@inbounds ai = Aip[h_start:h_end, w_start:w_end, ci, mi]
if cLayer isa MaxPoolLayer
mask = (Ao[hi,wi,ci,mi] .== ai)
@inbounds dAip[h_start:h_end, w_start:w_end, ci, mi] .+=
(dAo[hi, wi, ci, mi] .* mask)
else
mask = similar(ai)
mask .= 1 / prod(size(ai))
@inbounds dAip[h_start:h_end, w_start:w_end, ci, mi] .+=
(dAo[hi, wi, ci, mi] .* mask)
end #if cLayer isa MaxPoolLayer
end #for wi=1:n_W, hi=1:n_H
end #for ci=1:c
end #for mi=1:m
# end #if sS == fS
dAi .= dAip[1+padS[1]:end-padS[2], 1+padS[3]:end-padS[4], :, :]
return nothing
end #function dpooling!(cLayer::TwoD,
function dpooling!(
cLayer::ThreeD,
Ai::AbstractArray{T,5},
dAi::AbstractArray{T,5},
Ao::AbstractArray{T,5},
dAo::AbstractArray{T,5},
) where {ThreeD<:Union{MaxPool3D,AveragePool3D},T}
padS = paddingSize(cLayer, Ai)
paddedS = paddedSize(cLayer, Ai)
Aip = padding(cLayer, Ai)
dAip = zeros(eltype(Ai), paddedS)
n_Hi, n_Wi, n_Di, c, m = paddedS
s_H, s_W, s_D = sS = cLayer.s
f_H, f_W, f_D = fS = cLayer.f
n_H = (n_Hi - f_H) ÷ s_H + 1
n_W = (n_Wi - f_W) ÷ s_W + 1
n_D = (n_Di - f_D) ÷ s_D + 1
# if sS == fS
# # @simd
# for mi = 1:m,
# # @simd for
# ci = 1:c,
# # @simd for
# di = 1:n_D,
# # @simd for
# wi = 1:n_W
# # @simd
# Threads.@threads for hi = 1:n_H
# h_start = hi * s_H - (s_H == 1 ? 0 : s_H - 1)
# h_end =
# hi * s_H - (s_H == 1 ? 0 : s_H - 1) + f_H - 1
# w_start = wi * s_W - (s_W == 1 ? 0 : s_W - 1)
# w_end =
# wi * s_W - (s_W == 1 ? 0 : s_W - 1) + f_W - 1
# d_start = di * s_D - (s_D == 1 ? 0 : s_D - 1)
# d_end =
# di * s_D - (s_D == 1 ? 0 : s_D - 1) + f_D - 1
# @inbounds ai = Aip[
# h_start:h_end,
# w_start:w_end,
# d_start:d_end,
# ci,
# mi,
# ]
# if cLayer isa MaxPoolLayer
# mask = (Ao[hi,wi,di,ci,mi] .== ai)
# @inbounds dAip[
# h_start:h_end,
# w_start:w_end,
# d_start:d_end,
# ci,
# mi,
# ] .+= (dAo[hi, wi, di, ci, mi] .* mask)
# else
# mask = similar(ai)
# mask .= 1 / prod(size(ai))
# @inbounds dAip[
# h_start:h_end,
# w_start:w_end,
# d_start:d_end,
# ci,
# mi,
# ] .+= (dAo[hi, wi, di, ci, mi] .* mask)
# end #if cLayer isa MaxPoolLayer
# end #for hi=1:n_H
# # end #for wi=1:n_W
# # end #for di=1:n_D
# # end #for ci=1:c
# end #for mi=1:m
#
# else
# @simd
for mi = 1:m
# @simd
Threads.@threads for ci = 1:c
for di = 1:n_D, wi = 1:n_W, hi = 1:n_H
h_start = hi * s_H - (s_H == 1 ? 0 : s_H - 1)
h_end = hi * s_H - (s_H == 1 ? 0 : s_H - 1) + f_H - 1
w_start = wi * s_W - (s_W == 1 ? 0 : s_W - 1)
w_end = wi * s_W - (s_W == 1 ? 0 : s_W - 1) + f_W - 1
d_start = di * s_D - (s_D == 1 ? 0 : s_D - 1)
d_end = di * s_D - (s_D == 1 ? 0 : s_D - 1) + f_D - 1
@inbounds ai =
Aip[h_start:h_end, w_start:w_end, d_start:d_end, ci, mi]
if cLayer isa MaxPoolLayer
mask = (Ao[hi,wi,di,ci,mi] .== ai)
@inbounds dAip[
h_start:h_end,
w_start:w_end,
d_start:d_end,
ci,
mi,
] .+= (dAo[hi, wi, di, ci, mi] .* mask)
else
mask = similar(ai)
mask .= 1 / prod(size(ai))
@inbounds dAip[
h_start:h_end,
w_start:w_end,
d_start:d_end,
ci,
mi,
] .+= (dAo[hi, wi, di, ci, mi] .* mask)
end #if cLayer isa MaxPoolLayer
end #for wi=1:n_W, hi=1:n_H, di=1:n_D
end #for ci=1:c
end #for mi=1:m
# end #if sS == fS
dAi .= dAip[1+padS[1]:end-padS[2],
1+padS[3]:end-padS[4],
1+padS[5]:end-padS[6],
:,
:,
]
return nothing
end #function dpooling!(cLayer::ThreeD,
export dpooling!
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 14125 | using Statistics
function pooling!(
cLayer::OneD,
Ai::AbstractArray{T,3},
) where {OneD<:Union{MaxPool1D,AveragePool1D},T}
Aip = padding(cLayer, Ai)
n_Hi, c, m = size(Aip)
s_H = cLayer.s
f_H = cLayer.f
n_H = (n_Hi - f_H) ÷ s_H + 1
if cLayer isa MaxPoolLayer
pool = maximum
else
pool = mean
end #if cLayer isa MaxPoolLayer
@simd for mi = 1:m
@simd for ci = 1:c
@simd for hi = 1:n_H
h_start = hi * s_H - (s_H == 1 ? 0 : s_H - 1)
h_end = hi * s_H - (s_H == 1 ? 0 : s_H - 1) + f_H - 1
@inbounds ai = Aip[h_start:h_end, ci, mi]
@inbounds cLayer.A[hi, ci, mi] = pool(ai)
# ai = nothing
# Base.GC.gc()
end #for
end #for ci=1:c
end #for mi=1:m, ci=1:c
return nothing
end #function pooling!(cLayer::OneD
function pooling!(
cLayer::TwoD,
Ai::AbstractArray{T,4},
) where {TwoD<:Union{MaxPool2D,AveragePool2D},T}
Aip = padding(cLayer, Ai)
n_Hi, n_Wi, c, m = size(Aip)
s_H, s_W = cLayer.s
f_H, f_W = cLayer.f
n_H = (n_Hi - f_H) ÷ s_H + 1
n_W = (n_Wi - f_W) ÷ s_W + 1
if cLayer isa MaxPoolLayer
pool = maximum
else
pool = mean
end #if cLayer isa MaxPoolLayer
@simd for mi = 1:m
@simd for ci = 1:c
@simd for wi = 1:n_W
@simd for hi = 1:n_H
h_start = hi * s_H - (s_H == 1 ? 0 : s_H - 1)
h_end = hi * s_H - (s_H == 1 ? 0 : s_H - 1) + f_H - 1
w_start = wi * s_W - (s_W == 1 ? 0 : s_W - 1)
w_end = wi * s_W - (s_W == 1 ? 0 : s_W - 1) + f_W - 1
@inbounds ai =
view(Aip, h_start:h_end, w_start:w_end, ci, mi)
@inbounds cLayer.A[hi, wi, ci, mi] = pool(ai)
# ai = nothing
# Base.GC.gc()
end #for hi=1:n_H
end #for wi=1:n_W
end #for ci=1:c
end #for mi=1:m,
return nothing
end #function pooling!(cLayer::TwoD,
function pooling!(
cLayer::ThreeD,
Ai::AbstractArray{T,5},
) where {ThreeD<:Union{MaxPool3D,AveragePool3D},T}
Aip = padding(cLayer, Ai)
n_Hi, n_Wi, n_Di, c, m = size(Aip)
s_H, s_W, s_D = cLayer.s
f_H, f_W, f_D = cLayer.f
n_H = (n_Hi - f_H) ÷ s_H + 1
n_W = (n_Wi - f_W) ÷ s_W + 1
n_D = (n_Di - f_D) ÷ s_D + 1
if cLayer isa MaxPoolLayer
pool = maximum
else
pool = mean
end #if cLayer isa MaxPoolLayer
@simd for mi = 1:m
@simd for ci = 1:c
@simd for di = 1:n_D
@simd for wi = 1:n_W
@simd for hi = 1:n_H
h_start = hi * s_H - (s_H == 1 ? 0 : s_H - 1)
h_end = hi * s_H - (s_H == 1 ? 0 : s_H - 1) + f_H - 1
w_start = wi * s_W - (s_W == 1 ? 0 : s_W - 1)
w_end = wi * s_W - (s_W == 1 ? 0 : s_W - 1) + f_W - 1
d_start = di * s_D - (s_D == 1 ? 0 : s_D - 1)
d_end = di * s_D - (s_D == 1 ? 0 : s_D - 1) + f_D - 1
@inbounds ai = Aip[
h_start:h_end,
w_start:w_end,
d_start:d_end,
ci,
mi,
]
@inbounds cLayer.A[hi, wi, di, ci, mi] = pool(ai)
# ai = nothing
# Base.GC.gc()
end #for hi=1:n_H
end #for wi=1:n_W
end #for di=1:n_D
end #for ci=1:c
end #for mi=1:m, ci=1:c
return nothing
end #function pooling!(cLayer::ThreeD,
export pooling!
###dpooling
function dpooling!(
cLayer::OneD,
Ai::AbstractArray{T,3},
dAi::AbstractArray{T,3},
Ao::AbstractArray{T,3},
dAo::AbstractArray{T,3},
) where {OneD<:Union{MaxPool1D,AveragePool1D},T}
padS = paddingSize(cLayer, Ai)
paddedS = paddedSize(cLayer, Ai)
dAip = zeros(eltype(Ai), paddedS)
n_Hi, c, m = paddedS
s_H = sS = cLayer.s
f_H = fS = cLayer.f
n_H = (n_Hi - f_H) ÷ s_H + 1
if sS == fS #to use the @simd the parallele the operation
@simd for mi = 1:m
@simd for ci = 1:c
@simd for hi = 1:n_H
h_start = hi * s_H - (s_H == 1 ? 0 : s_H - 1)
h_end = hi * s_H - (s_H == 1 ? 0 : s_H - 1) + f_H - 1
@inbounds ai = Aip[h_start:h_end, ci, mi]
if cLayer isa MaxPoolLayer
mask = (Ao[hi,ci,mi] .== ai)
@inbounds dAip[h_start:h_end, ci, mi] .+=
(dAo[hi, ci, mi] .* mask)
else
mask = similar(ai)
mask .= 1 / prod(size(ai))
@inbounds dAip[h_start:h_end, ci, mi] .+=
(dAo[hi, ci, mi] .* mask)
end #if cLayer isa MaxPoolLayer
end #for hi=1:n_H
end #for ci=1:c
end #for mi=1:m
else
@simd for mi = 1:m
@simd for ci = 1:c
for hi = 1:n_H
h_start = hi * s_H - (s_H == 1 ? 0 : s_H - 1)
h_end = hi * s_H - (s_H == 1 ? 0 : s_H - 1) + f_H - 1
@inbounds ai = Aip[h_start:h_end, ci, mi]
if cLayer isa MaxPoolLayer
mask = (Ao[hi,ci,mi] .== ai)
@inbounds dAip[h_start:h_end, ci, mi] .+=
(dAo[hi, ci, mi] .* mask)
else
mask = similar(ai)
mask .= 1 / prod(size(ai))
@inbounds dAip[h_start:h_end, ci, mi] .+=
(dAo[hi, ci, mi] .* mask)
end #if cLayer isa MaxPoolLayer
end #for hi=1:n_H
end #for ci=1:c
end #for mi=1:m
end #if sS == fS
dAi .= dAip[1+padS[1]:end-padS[2], :, :]
return nothing
end #function dpooling!(cLayer::OneD
function dpooling!(
cLayer::TwoD,
Ai::AbstractArray{T,4},
dAi::AbstractArray{T,4},
Ao::AbstractArray{T,4},
dAo::AbstractArray{T,4},
) where {TwoD<:Union{MaxPool2D,AveragePool2D},T}
padS = paddingSize(cLayer, Ai)
paddedS = paddedSize(cLayer, Ai)
dAip = zeros(eltype(Ai), paddedS)
n_Hi, n_Wi, c, m = paddedS
s_H, s_W = sS = cLayer.s
f_H, f_W = fS = cLayer.f
n_H = (n_Hi - f_H) ÷ s_H + 1
n_W = (n_Wi - f_W) ÷ s_W + 1
if sS == fS
@simd for mi = 1:m
@simd for ci = 1:c
@simd for wi = 1:n_W
@simd for hi = 1:n_H
h_start = hi * s_H - (s_H == 1 ? 0 : s_H - 1)
h_end = hi * s_H - (s_H == 1 ? 0 : s_H - 1) + f_H - 1
w_start = wi * s_W - (s_W == 1 ? 0 : s_W - 1)
w_end = wi * s_W - (s_W == 1 ? 0 : s_W - 1) + f_W - 1
@inbounds ai = Aip[h_start:h_end, w_start:w_end, ci, mi]
if cLayer isa MaxPoolLayer
mask = (Ao[hi,wi,ci,mi] .== ai)
@inbounds dAip[
h_start:h_end,
w_start:w_end,
ci,
mi,
] .+= (dAo[hi, wi, ci, mi] .* mask)
else
mask = similar(ai)
mask .= 1 / prod(size(ai))
@inbounds dAip[
h_start:h_end,
w_start:w_end,
ci,
mi,
] .+= (dAo[hi, wi, ci, mi] .* mask)
end #if cLayer isa MaxPoolLayer
end #for hi=1:n_H
end #for wi=1:n_W
end #for ci=1:c
end #for mi=1:m
else
@simd for mi = 1:m
@simd for ci = 1:c
for wi = 1:n_W, hi = 1:n_H
h_start = hi * s_H - (s_H == 1 ? 0 : s_H - 1)
h_end = hi * s_H - (s_H == 1 ? 0 : s_H - 1) + f_H - 1
w_start = wi * s_W - (s_W == 1 ? 0 : s_W - 1)
w_end = wi * s_W - (s_W == 1 ? 0 : s_W - 1) + f_W - 1
@inbounds ai = Aip[h_start:h_end, w_start:w_end, ci, mi]
if cLayer isa MaxPoolLayer
mask = (Ao[hi,wi,ci,mi] .== ai)
@inbounds dAip[h_start:h_end, w_start:w_end, ci, mi] .+=
(dAo[hi, wi, ci, mi] .* mask)
else
mask = similar(ai)
mask .= 1 / prod(size(ai))
@inbounds dAip[h_start:h_end, w_start:w_end, ci, mi] .+=
(dAo[hi, wi, ci, mi] .* mask)
end #if cLayer isa MaxPoolLayer
end #for wi=1:n_W, hi=1:n_H
end #for ci=1:c
end #for mi=1:m
end #if sS == fS
dAi .= dAip[1+padS[1]:end-padS[2], 1+padS[3]:end-padS[4], :, :]
return nothing
end #function dpooling!(cLayer::TwoD,
function dpooling!(
cLayer::ThreeD,
Ai::AbstractArray{T,5},
dAi::AbstractArray{T,5},
Ao::AbstractArray{T,5},
dAo::AbstractArray{T,5},
) where {ThreeD<:Union{MaxPool3D,AveragePool3D},T}
padS = paddingSize(cLayer, Ai)
paddedS = paddedSize(cLayer, Ai)
dAip = zeros(eltype(Ai), paddedS)
n_Hi, n_Wi, n_Di, c, m = paddedS
s_H, s_W, s_D = sS = cLayer.s
f_H, f_W, f_D = fS = cLayer.f
n_H = (n_Hi - f_H) ÷ s_H + 1
n_W = (n_Wi - f_W) ÷ s_W + 1
n_D = (n_Di - f_D) ÷ s_D + 1
if sS == fS
@simd for mi = 1:m
@simd for ci = 1:c
@simd for di = 1:n_D
@simd for wi = 1:n_W
@simd for hi = 1:n_H
h_start = hi * s_H - (s_H == 1 ? 0 : s_H - 1)
h_end =
hi * s_H - (s_H == 1 ? 0 : s_H - 1) + f_H - 1
w_start = wi * s_W - (s_W == 1 ? 0 : s_W - 1)
w_end =
wi * s_W - (s_W == 1 ? 0 : s_W - 1) + f_W - 1
d_start = di * s_D - (s_D == 1 ? 0 : s_D - 1)
d_end =
di * s_D - (s_D == 1 ? 0 : s_D - 1) + f_D - 1
@inbounds ai = Aip[
h_start:h_end,
w_start:w_end,
d_start:d_end,
ci,
mi,
]
if cLayer isa MaxPoolLayer
mask = (Ao[hi,wi,di,ci,mi] .== ai)
@inbounds dAip[
h_start:h_end,
w_start:w_end,
d_start:d_end,
ci,
mi,
] .+= (dAo[hi, wi, di, ci, mi] .* mask)
else
mask = similar(ai)
mask .= 1 / prod(size(ai))
@inbounds dAip[
h_start:h_end,
w_start:w_end,
d_start:d_end,
ci,
mi,
] .+= (dAo[hi, wi, di, ci, mi] .* mask)
end #if cLayer isa MaxPoolLayer
end #for hi=1:n_H
end #for wi=1:n_W
end #for di=1:n_D
end #for ci=1:c
end #for mi=1:m
else
@simd for mi = 1:m
@simd for ci = 1:c
for di = 1:n_D, wi = 1:n_W, hi = 1:n_H
h_start = hi * s_H - (s_H == 1 ? 0 : s_H - 1)
h_end = hi * s_H - (s_H == 1 ? 0 : s_H - 1) + f_H - 1
w_start = wi * s_W - (s_W == 1 ? 0 : s_W - 1)
w_end = wi * s_W - (s_W == 1 ? 0 : s_W - 1) + f_W - 1
d_start = di * s_D - (s_D == 1 ? 0 : s_D - 1)
d_end = di * s_D - (s_D == 1 ? 0 : s_D - 1) + f_D - 1
@inbounds ai =
Aip[h_start:h_end, w_start:w_end, d_start:d_end, ci, mi]
if cLayer isa MaxPoolLayer
mask = (Ao[hi,wi,di,ci,mi] .== ai)
@inbounds dAip[
h_start:h_end,
w_start:w_end,
d_start:d_end,
ci,
mi,
] .+= (dAo[hi, wi, di, ci, mi] .* mask)
else
mask = similar(ai)
mask .= 1 / prod(size(ai))
@inbounds dAip[
h_start:h_end,
w_start:w_end,
d_start:d_end,
ci,
mi,
] .+= (dAo[hi, wi, di, ci, mi] .* mask)
end #if cLayer isa MaxPoolLayer
end #for wi=1:n_W, hi=1:n_H, di=1:n_D
end #for ci=1:c
end #for mi=1:m
end #if sS == fS
dAi .= dAip[1+padS[1]:end-padS[2],
1+padS[3]:end-padS[4],
1+padS[5]:end-padS[6],
:,
:,
]
return nothing
end #function dpooling!(cLayer::ThreeD,
export dpooling!
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 8961 | using PaddedViews
#TODO use parallel processing to speed up the unrolling process
#TODO build a reroll function to extract W from K
### unroll conv1d
@doc raw"""
unroll(cLayer::Conv1D, AiS::Tuple, param::Symbol=:W)
unroll the `param` of `Conv1D` into 2D matrix
# Arguments
- `cLayer` := the layer of the paramters to unroll
- `AiS` := the `padded` input to determinde the size and shape of the output of `unroll`
- `param` := `Conv1D` parameter to be `unroll`ed
# Return
- `K` := 2D `Matrix` of the `param`
"""
function unroll(cLayer::Conv1D, AiS::Tuple, param::Symbol=:W)
prevLayer = cLayer.prevLayer
n_Hi, ci, m = AiS
f_H = cLayer.f
s_H = cLayer.s
c = cLayer.channels
n_H = (n_Hi - f_H) ÷ s_H + 1
W = eval(:($cLayer.$param))
T = eltype(W)
B = cLayer.B
K = nothing
for ch=1:size(W)[end]
k2d = nothing
w3d = W[:,:,ch]
k = nothing
for i=range(1, step=s_H, length=n_H)
w2d = PaddedView(0, w3d, (n_Hi,ci), (i,1))
for w=1:size(w2d)[2]
if w==1
k2d = w2d[:,w]
else
k2d = vcat(k2d, w2d[:,w])
end #if h==1
end #for w=1:size(w3d)[3]
if i==1
k = k2d
else
k = hcat(k, k2d)
end #if i==1
end #for i=1:n_W
if ch == 1
K = k
else
K = hcat(K, k)
end #if ch==1
end #for ch=1:size(W)[end]
return transpose(K)
end #function unroll(cLayer::Conv2D)
###unroll conv2d
@doc raw"""
unroll(cLayer::Conv2D, AiS::Tuple, param::Symbol=:W)
unroll the `param` of `Conv1D` into 2D matrix
# Arguments
- `cLayer` := the layer of the paramters to unroll
- `AiS` := the `padded` input to determinde the size and shape of the output of `unroll`
- `param` := `Conv1D` parameter to be `unroll`ed
# Return
- `K` := 2D `Matrix` of the `param`
"""
function unroll(cLayer::Conv2D, AiS::Tuple, param::Symbol=:W)
prevLayer = cLayer.prevLayer
n_Hi, n_Wi, ci, m = AiS
HWi = n_Hi*n_Wi
f_H, f_W = cLayer.f
fHW = f_H * f_W
s_H, s_W = cLayer.s
c = cLayer.channels
n_H = (n_Hi - f_H) ÷ s_H + 1
n_W = (n_Wi - f_W) ÷ s_W + 1
W = eval(:($cLayer.$param))
T = eltype(W)
B = cLayer.B
K = nothing
for ch=1:size(W)[end]
w3da = W[:,:,:,ch]
k2d = nothing
k3d = nothing
k4d = nothing
k = nothing
for i=range(1, step=s_H, length=n_H)
w3db = PaddedView(0, w3da, (n_Hi, f_W,ci), (i,1,1))
for j=range(1, step=s_W, length=n_W)
w3d = PaddedView(0, w3db, (n_Hi, n_Wi,ci), (1,j,1))
for w=1:size(w3d)[3]
for h=1:size(w3d)[1]
if h==1
k2d = w3d[h,:,w]
else
k2d = vcat(k2d, w3d[h,:,w])
end #if h==1
end #for h=1:size(w)[1]
if w==1
k3d = k2d
else
k3d = vcat(k3d, k2d)
end #if w==1
end #for w=1:size(w3d)[3]
if j==1
k4d = k3d
else
k4d = hcat(k4d, k3d)
end
end #for j=1:n_H
if i==1
k = k4d
else
k = hcat(k, k4d)
end #if i==1
end #for i=1:n_W
if ch==1
K = k
else
K = hcat(K, k)
end #if ch==1
end #for ch=1:size(W)[end]
return transpose(K)
end #function unroll(cLayer::Conv2D)
###unroll conv3d
@doc raw"""
unroll(cLayer::Conv3D, AiS::Tuple, param::Symbol=:W)
unroll the `param` of `Conv3D` into 2D matrix
# Arguments
- `cLayer` := the layer of the paramters to unroll
- `AiS` := the `padded` input to determinde the size and shape of the output of `unroll`
- `param` := `Conv1D` parameter to be `unroll`ed
# Return
- `K` := 2D `Matrix` of the `param`
"""
function unroll(cLayer::Conv3D, AiS::Tuple, param::Symbol=:W)
prevLayer = cLayer.prevLayer
n_Hi, n_Wi, n_Di, ci, m = AiS
HWDi = n_Hi*n_Wi*n_Di
f_H, f_W, f_D = cLayer.f
fHW = f_H * f_W * f_D
s_H, s_W, s_D = cLayer.s
c = cLayer.channels
n_H = (n_Hi - f_H) ÷ s_H + 1
n_W = (n_Wi - f_W) ÷ s_W + 1
n_D = (n_Di - f_D) ÷ s_D + 1
W = eval(:($cLayer.$param))
T = eltype(W)
B = cLayer.B
K = nothing
for ch=1:size(W)[end]
w4da = W[:,:,:,:,ch]
k2d = nothing
k3d = nothing
k4d = nothing
k5d = nothing
k = nothing
k1 = nothing
for l=range(1, step=s_D, length=n_D)
w4db = PaddedView(0, w4da, (f_H, f_W, n_Di, ci), (1,1,l,1))
for i=range(1, step=s_H, length=n_H)
w4dc = PaddedView(0, w4db, (n_Hi, f_W, n_Di, ci), (i,1,1,1))
for j=range(1, step=s_W, length=n_W)
w4d = PaddedView(0, w4dc, (n_Hi, n_Wi, n_Di, ci), (1,j,1,1))
for w=1:size(w4d)[4]
for d=1:size(w4d)[3]
for h=1:size(w4d)[1]
if h==1
k2d = w4d[h,:,d,w]
else
k2d = vcat(k2d, w4d[h,:,d,w])
end #if h==1
end #for h=1:size(w)[1]
if d==1
k3d = deepcopy(k2d)
else
k3d = vcat(k3d, k2d)
end #if w==1
end #for w=1:size(w3d)[3]
if w==1
k4d = deepcopy(k3d)
else
k4d = vcat(k4d, k3d)
end #if d==1
end #for d=1:size(w4d)[4]
if j==1
k5d = deepcopy(k4d)
else
k5d = hcat(k5d, k4d)
end
end #for j=1:n_H
if i==1
k = deepcopy(k5d)
else
k = hcat(k, k5d)
end #if i==1
end #for i=1:n_W
if l==1
k1 = deepcopy(k)
else
k1 = hcat(k1, k)
end #if l==1
end #for l=range(1, step=s_D, length=n_D)
if ch==1
K = k1
else
K = hcat(K, k1)
end #if ch==1
end #for ch=1:size(W)[end]
return transpose(K)
end #function unroll(cLayer::Conv2D)
export unroll
function unrollcol(cLayer::Conv2D, AiS::Tuple, param::Symbol=:W)
prevLayer = cLayer.prevLayer
n_Hi, n_Wi, ci, m = AiS
HWi = n_Hi*n_Wi
f_H, f_W = cLayer.f
fHW = f_H * f_W
s_H, s_W = cLayer.s
c = cLayer.channels
n_H = (n_Hi - f_H) ÷ s_H + 1
n_W = (n_Wi - f_W) ÷ s_W + 1
W = eval(:($cLayer.$param))
T = eltype(W)
B = cLayer.B
K = nothing
for ch=1:size(W)[end]
w3da = W[:,:,:,ch]
k2d = nothing
k3d = nothing
k4d = nothing
k = nothing
for i=range(1, step=s_W, length=n_W)
w3db = PaddedView(0, w3da, (f_H, n_Wi,ci), (1,i,1))
for j=range(1, step=s_H, length=n_H)
w3d = PaddedView(0, w3db, (n_Hi, n_Wi,ci), (j,1,1))
for cj=1:size(w3d)[3]
for w=1:size(w3d)[2]
if w==1
k2d = w3d[:,w,cj]
else
k2d = vcat(k2d, w3d[:,w,cj])
end #if h==1
end #for h=1:size(w)[1]
if cj==1
k3d = k2d
k2d = nothing
else
k3d = vcat(k3d, k2d)
k2d = nothing
end #if w==1
end #for w=1:size(w3d)[3]
if j==1
k4d = k3d
k3d = nothing
else
k4d = hcat(k4d, k3d)
k3d = nothing
end
end #for j=1:n_H
if i==1
k = k4d
k4d = nothing
else
k = hcat(k, k4d)
k4d = nothing
end #if i==1
end #for i=1:n_W
if ch==1
K = k
k = nothing
Base.GC.gc()
else
K = hcat(K, k)
k2d = nothing
Base.GC.gc()
end #if ch==1
end #for ch=1:size(W)[end]
return transpose(K)
end #function unroll(cLayer::Conv2D)
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | code | 31 | using Test
@test true == true
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | docs | 2998 | GitHub main.yml Status | Travis CI building Status | Stable Documentation | Dev Documentation
----------|-----------|----------|-----------
 | [](https://travis-ci.com/MohHizzani/NumNN.jl) | [](https://mohhizzani.github.io/NumNN.jl/stable) | [](https://mohhizzani.github.io/NumNN.jl/dev)
# NumNN.jl
This package provides high-level Neural Network APIs deals with different number representations like [Posit][1], Logarithmic Data Representations, Residual Number System (RNS), and -for sure- the conventional IEEE formats.
Since, the implementation and development process for testing novel number systems on different Deep Learning applications using the current available DP frameworks in easily feasible. An urgent need for an unconventional library that provides both the easiness and complexity of simulating and testing and evaluate new number systems before the hardware design complex process— was resurfaced.
## Why Julia?
[Julia][2] provides in an unconventional way the ability to simulate new number systems and deploy this simulation to be used as high-level primitive type. **[Multiple Dispatch][3]** provides a unique ability to write a general code then specify the implementation based on the type.
### Examples of Multiple Dispatch
```julia
julia> aInt = 1; #with Integer type
julia> bInt = 2; #with Integer type
julia> cInt = 1 + 2; #which is a shortcut for cInt = +(1,2)
julia> aFloat = 1.0; #with Float64 type
julia> bFloat = 2.0; #with Flot64 type
julia> aInt + bFloat #will use the method +(::Int64, ::Float64)
3.0
```
Now let's do something more interesting with **Posit** (continue on the previous example)
```julia
julia> using SoftPosit
julia> aP = Posit16(1)
Posit16(0x4000)
julia> bP = Posit16(2)
Posit16(0x5000)
julia> aInt + aP #Note how the result is in Posit16 type
Posit16(0x5000)
```
The output was of type `Posit16` because in **Julia** you can define a [Promote Rule][4] which mean when the output can be in either type(s) of the input, **promote** the output to be of the specified type. Which can be defined as follows:
```julia
Base.promote_rule(::Type{Int64}, ::Type{Posit16}) = Posit16
```
This means that the output of an operation on both `Int64` and `Posit16` should be converted to `Posit16`.
## Install
To install in Julia
```julia
julia> ] add NumNN
```
## To Use
```julia
julia> using NumNN
```
[1]: <superfri.org/superfri/article/view/137> "Beating Floating Point at its Own Game: Posit Arithmetic"
[2]: <julialang.org> "Julia Language"
[3]: <https://docs.julialang.org/en/v1/manual/methods/> "Julia Multiple Dispatch"
[4]: <https://docs.julialang.org/en/v1/manual/conversion-and-promotion/#Promotion-1> "Juila Promotion"
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | docs | 80 | # Docstrings
```@autodocs
Modules = [NumNN]
Order = [:type, :function]
```
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | docs | 2356 | # NumNN.jl
This package provides high-level Neural Network APIs deals with different number representations like [Posit](https://www.superfri.org/superfri/article/view/137 "Beating Floating Point at its Own Game: Posit Arithmetic"), Logarithmic Data Representations, Residual Number System (RNS), and -for sure- the conventional IEEE formats.
Since, the implementation and development process for testing novel number systems on different Deep Learning applications using the current available DP frameworks in easily feasible. An urgent need for an unconventional library that provides both the easiness and complexity of simulating and testing and evaluate new number systems before the hardware design complex process— was resurfaced.
## Why Julia?
[Julia](https://julialang.org/ "Julia Language") provides in an unconventional way the ability to simulate new number systems and deploy this simulation to be used as high-level primitive type. **[Multiple Dispatch](https://docs.julialang.org/en/v1/manual/methods/ "Julia Multiple Dispatch")** provides a unique ability to write a general code then specify the implementation based on the type.
### Examples of Multiple Dispatch
```julia-repl
julia> aInt = 1; #with Integer type
julia> bInt = 2; #with Integer type
julia> cInt = 1 + 2; #which is a shortcut for cInt = +(1,2)
julia> aFloat = 1.0; #with Float64 type
julia> bFloat = 2.0; #with Flot64 type
julia> aInt + bFloat #will use the method +(::Int64, ::Float64)
3.0
```
Now let's do something more interesting with **Posit** (continue on the previous example)
```julia-repl
julia> using SoftPosit
julia> aP = Posit16(1)
Posit16(0x4000)
julia> bP = Posit16(2)
Posit16(0x5000)
julia> aInt + aP #Note how the result is in Posit16 type
Posit16(0x5000)
```
The output was of type `Posit16` because in **Julia** you can define a [Promote Rule](https://docs.julialang.org/en/v1/manual/conversion-and-promotion/#Promotion-1 "Juila Promotion") which mean when the output can be in either type(s) of the input, **promote** the output to be of the specified type. Which can be defined as follows:
```julia
Base.promote_rule(::Type{Int64}, ::Type{Posit16}) = Posit16
```
This means that the output of an operation on both `Int64` and `Posit16` should be converted to `Posit16`.
```@contents
Pages = ["docstrings.md", "tutorials.md"]
```
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.5.3 | a1f51078c01785207ec90a733bbceff8c2ecbe72 | docs | 633 | # Tutorials
To learn how to use [NumNN](https://github.com/MohHizzani/NumNN.jl), here some tutorials as [Jupyter](https://jupyter.org/) notebooks
## [Intro to **NumNN** using Fully-Connected Layers](https://nbviewer.jupyter.org/github/MohHizzani/NumNN.jl/blob/master/examples/00_FCLayer_FashionMNIST.ipynb)
## [Intro to **NumNN** using Convolution Neural Networks](https://nbviewer.jupyter.org/github/MohHizzani/NumNN.jl/blob/master/examples/01_CNN_FashionMNIST.ipynb)
## [Example of **Inception** Neural Network](https://nbviewer.jupyter.org/github/MohHizzani/NumNN.jl/blob/master/examples/02_CNN_Inception_FashionMNIST.ipynb)
| NumNN | https://github.com/MohHizzani/NumNN.jl.git |
|
[
"MIT"
] | 0.1.9 | fb00568e77176ce5a5e9266277b7a696cbc4e0f3 | code | 878 | module ConstraintLearning
# SECTION - imports
using ConstraintDomains
using Constraints
using LocalSearchSolvers
using CompositionalNetworks
using Dictionaries
using Evolutionary
using Memoization
using TestItems
using ThreadPools
using QUBOConstraints
using DataFrames
using Flux
using PrettyTables
import Flux.Optimise: update!
import Flux: params
import CompositionalNetworks: exclu, nbits_exclu, nbits, layers, compose, as_int
# SECTION - exports
export icn
export qubo
export ICNConfig
export ICNGeneticOptimizer
export ICNLocalSearchOptimizer
export ICNOptimizer
export QUBOGradientOptimizer
export QUBOOptimizer
# SECTION - includes common
include("common.jl")
# SECTION - ICN
include("icn/base.jl")
include("icn/genetic.jl")
include("icn/cbls.jl")
include("icn.jl")
# SECTION - QUBO
include("qubo/base.jl")
include("qubo/gradient.jl")
include("qubo.jl")
end
| ConstraintLearning | https://github.com/JuliaConstraints/ConstraintLearning.jl.git |
|
[
"MIT"
] | 0.1.9 | fb00568e77176ce5a5e9266277b7a696cbc4e0f3 | code | 2025 | """
δ(X[, Y]; discrete = true)
Compute the extrema over a collection `X`` or a pair of collection `(X, Y)`.
"""
function δ(X; discrete = true)
mn, mx = extrema(X)
return mx - mn + discrete
end
function δ(X, Y; discrete = true)
mnx, mxx = extrema(X)
mny, mxy = extrema(Y)
return max(mxx, mxy) - min(mnx, mny) + discrete
end
"""
sub_eltype(X)
Return the element type of of the first element of a collection.
"""
sub_eltype(X) = eltype(first(T))
"""
domain_size(ds::Number)
Extends the domain_size function when `ds` is number (for dispatch purposes).
"""
domain_size(ds::Number) = ds
"""
make_training_sets(X, penalty, args...)
Return a pair of solutions and non solutions sets based on `X` and `penalty`.
"""
function make_training_sets(X, penalty, p, ds)
f = isnothing(p) ? ((x; param = p) -> penalty(x)) : penalty
solutions = Set{Vector{Int}}()
non_sltns = Set{Vector{Int}}()
foreach(
c -> (cv = collect(c); push!(f(cv; param = p) ? solutions : non_sltns, cv)),
X
)
return solutions, non_sltns
end
# REVIEW - Is it correct? Make a CI test
function make_training_sets(X, penalty::Vector{T}, _) where {T <: Real}
solutions = Set{Vector{Int}}()
non_sltns = Set{Vector{Int}}()
foreach(
(c, p) -> (cv = collect(c); push!(p ? non_sltns : solutions, cv)),
Iterators.zip(X, penalty)
)
return solutions, non_sltns
end
"""
make_set_penalty(X, X̅, args...; kargs)
Return a penalty function when the training set is already split into a pair of solutions `X` and non solutions `X̅`.
"""
function make_set_penalty(X, X̅)
penalty = x -> x ∈ X ? 1.0 : x ∈ X̅ ? 0.0 : 0.5
X_train = union(X, X̅)
return X_train, penalty
end
make_set_penalty(X, X̅, ::Nothing) = make_set_penalty(X, X̅)
function make_set_penalty(X, X̅, icn_conf; parameters...)
penalty = icn(X, X̅; metric = icn_conf.metric, optimizer = icn.optimizer, parameters...)
X_train = union(X, X̅)
return X_train, penalty
end
| ConstraintLearning | https://github.com/JuliaConstraints/ConstraintLearning.jl.git |
|
[
"MIT"
] | 0.1.9 | fb00568e77176ce5a5e9266277b7a696cbc4e0f3 | code | 1811 | """
icn(X,X̅; kargs..., parameters...)
TBW
"""
function icn(
X,
X̅;
discrete = true,
dom_size = δ(Iterators.flatten(X), Iterators.flatten(X̅); discrete),
metric = :hamming,
optimizer = ICNGeneticOptimizer(),
X_test = nothing,
parameters...
)
lc = learn_compose(
X,
X̅,
dom_size;
metric,
optimizer,
X_test,
parameters...
)[1]
return composition(lc)
end
function icn(
domains::Vector{D},
penalty::F;
configurations = nothing,
discrete = true,
dom_size = nothing,
metric = :hamming,
optimizer = ICNGeneticOptimizer(),
X_test = nothing,
parameters...
) where {D <: AbstractDomain, F <: Function}
if isnothing(configurations)
configurations = explore(domains, penalty; parameters...)
end
if isnothing(dom_size)
dom_size = δ(
Iterators.flatten(configurations[1]),
Iterators.flatten(configurations[2]);
discrete
)
end
return icn(
configurations[1],
configurations[2];
discrete,
dom_size,
metric,
optimizer,
X_test,
parameters...
)
end
function icn(
X,
penalty::F;
discrete = true,
dom_size = δ(Iterators.flatten(X); discrete),
metric = :hamming,
optimizer = ICNGeneticOptimizer(),
X_test = nothing,
parameters...
) where {F <: Function}
solutions, non_sltns = make_training_sets(X, penalty, dom_size; parameters...)
return icn(
solutions,
non_sltns;
discrete,
dom_size,
metric,
optimizer,
X_test,
parameters...
)
end
| ConstraintLearning | https://github.com/JuliaConstraints/ConstraintLearning.jl.git |
|
[
"MIT"
] | 0.1.9 | fb00568e77176ce5a5e9266277b7a696cbc4e0f3 | code | 818 | """
qubo(X,X̅; kargs..., parameters...)
TBW
"""
function qubo(
X,
penalty::Function,
dom_stuff = nothing;
param = nothing,
icn_conf = nothing,
optimizer = GradientDescentOptimizer(),
X_test = X
)
if icn_conf !== nothing
penalty = icn(
X, penalty; param, metric = icn_conf.metric, optimizer = icn_conf.optimizer)
end
return train(X, penalty, dom_stuff; optimizer, X_test)
end
function qubo(
X,
X̅,
dom_stuff = nothing;
icn_conf = nothing,
optimizer = GradientDescentOptimizer(),
param = nothing,
X_test = union(X, X̅)
)
X_train, penalty = make_set_penalty(X, X̅, param, icn_conf)
return qubo(X_train, penalty, dom_stuff; icn_conf, optimizer, param, X_test)
end
| ConstraintLearning | https://github.com/JuliaConstraints/ConstraintLearning.jl.git |
|
[
"MIT"
] | 0.1.9 | fb00568e77176ce5a5e9266277b7a696cbc4e0f3 | code | 415 | """
const ICNOptimizer = CompositionalNetworks.AbstractOptimizer
An abstract type for optmizers defined to learn ICNs.
"""
const ICNOptimizer = CompositionalNetworks.AbstractOptimizer
"""
struct ICNConfig{O <: ICNOptimizer}
A structure to hold the metric and optimizer configurations used in learning the weights of an ICN.
"""
struct ICNConfig{O <: ICNOptimizer}
metric::Symbol
optimizer::O
end
| ConstraintLearning | https://github.com/JuliaConstraints/ConstraintLearning.jl.git |
|
[
"MIT"
] | 0.1.9 | fb00568e77176ce5a5e9266277b7a696cbc4e0f3 | code | 3172 | """
ICNLocalSearchOptimizer(options = LocalSearchSolvers.Options())
Default constructor to learn an ICN through a CBLS solver.
"""
struct ICNLocalSearchOptimizer <: ICNOptimizer
options::LocalSearchSolvers.Options
ICNLocalSearchOptimizer(options = LocalSearchSolvers.Options()) = new(options)
end
"""
mutually_exclusive(layer, w)
Constraint ensuring that `w` encode exclusive operations in `layer`.
"""
function mutually_exclusive(layer, w)
x = as_int(w)
l = length(layer)
return iszero(x) ? 1.0 : max(0.0, x - l)
end
"""
no_empty_layer(x; X = nothing)
Constraint ensuring that at least one operation is selected.
"""
no_empty_layer(x; X = nothing) = max(0, 1 - sum(x))
"""
parameter_specific_operations(x; X = nothing)
Constraint ensuring that at least one operation related to parameters is selected if the error function to be learned is parametric.
"""
parameter_specific_operations(x; X = nothing) = 0.0
"""
CompositionalNetworks.optimize!(icn, solutions, non_sltns, dom_size, metric, optimizer::ICNLocalSearchOptimizer; parameters...)
Extends the `optimize!` method to `ICNLocalSearchOptimizer`.
"""
function CompositionalNetworks.optimize!(
icn, solutions, non_sltns, dom_size, metric,
optimizer::ICNLocalSearchOptimizer; parameters...
)
@debug "starting debug opt"
m = model(; kind = :icn)
n = nbits(icn)
# All variables are boolean
d = domain([false, true])
# Add variables
foreach(_ -> variable!(m, d), 1:n)
# Add constraint
start = 1
for layer in layers(icn)
if exclu(layer)
stop = start + nbits_exclu(layer) - 1
f(x; X = nothing) = mutually_exclusive(layer, x)
constraint!(m, f, start:stop)
else
stop = start + length(layer) - 1
constraint!(m, no_empty_layer, start:stop)
end
start = stop + 1
end
# Add objective
inplace = zeros(dom_size, max_icn_length())
function fitness(w)
_w = BitVector(w)
compo = compose(icn, _w)
f = composition(compo)
S = Iterators.flatten((solutions, non_sltns))
@debug _w compo f S metric
σ = sum(
x -> abs(f(x; X = inplace, dom_size, parameters...) -
eval(metric)(x, solutions)),
S)
return σ + regularization(icn) + weights_bias(_w)
end
objective!(m, fitness)
# Create solver and solve
s = solver(m; options = optimizer.options)
solve!(s)
@debug "pool" s.pool best_values(s.pool) best_values(s) s.pool.configurations
# Return best values
if has_solution(s)
weights!(icn, BitVector(collect(best_values(s))))
else
CompositionalNetworks.generate_weights(icn)
end
best = weights(icn)
return best, Dictionary{BitVector, Int}([best], [1])
end
@testitem "ICN: CBLS" tags=[:icn, :cbls] default_imports=false begin
using ConstraintDomains
using ConstraintLearning
domains = [domain([1, 2, 3, 4]) for i in 1:4]
compo = icn(domains, allunique; optimizer = ICNLocalSearchOptimizer())
# @test compo([1,2,3,3], dom_size = 4) > 0.0
end
| ConstraintLearning | https://github.com/JuliaConstraints/ConstraintLearning.jl.git |
|
[
"MIT"
] | 0.1.9 | fb00568e77176ce5a5e9266277b7a696cbc4e0f3 | code | 4768 | """
generate_population(icn, pop_size
Generate a pôpulation of weights (individuals) for the genetic algorithm weighting `icn`.
"""
function generate_population(icn, pop_size)
population = Vector{BitVector}()
foreach(_ -> push!(population, falses(nbits(icn))), 1:pop_size)
return population
end
"""
_optimize!(icn, X, X_sols; metric = hamming, pop_size = 200)
Optimize and set the weights of an ICN with a given set of configuration `X` and solutions `X_sols`.
"""
function _optimize!(
icn,
solutions,
non_sltns,
dom_size,
metric,
pop_size,
iterations;
samples = nothing,
memoize = false,
parameters...
)
inplace = zeros(dom_size, max_icn_length())
_non_sltns = isnothing(samples) ? non_sltns : rand(non_sltns, samples)
function fitness(w)
compo = compose(icn, w)
f = composition(compo)
S = Iterators.flatten((solutions, _non_sltns))
σ = sum(
x -> abs(f(x; X = inplace, dom_size, parameters...) - metric(x, solutions)), S
)
return σ + regularization(icn) + weights_bias(w)
end
_fitness = memoize ? (@memoize Dict memoize_fitness(w)=fitness(w)) : fitness
_icn_ga = GA(;
populationSize = pop_size,
crossoverRate = 0.8,
epsilon = 0.05,
selection = tournament(2),
crossover = SPX,
mutation = flip,
mutationRate = 1.0
)
pop = generate_population(icn, pop_size)
r = Evolutionary.optimize(_fitness, pop, _icn_ga, Evolutionary.Options(; iterations))
return weights!(icn, Evolutionary.minimizer(r))
end
"""
optimize!(icn, X, X_sols, global_iter, local_iter; metric=hamming, popSize=100)
Optimize and set the weights of an ICN with a given set of configuration `X` and solutions `X_sols`. The best weights among `global_iter` will be set.
"""
function optimize!(
icn,
solutions,
non_sltns,
global_iter,
iter,
dom_size,
metric,
pop_size;
sampler = nothing,
memoize = false,
parameters...
)
results = Dictionary{BitVector, Int}()
aux_results = Vector{BitVector}(undef, global_iter)
nt = Base.Threads.nthreads()
@info """Starting optimization of weights$(nt > 1 ? " (multithreaded)" : "")"""
samples = isnothing(sampler) ? nothing : sampler(length(solutions) + length(non_sltns))
@qthreads for i in 1:global_iter
@info "Iteration $i"
aux_icn = deepcopy(icn)
_optimize!(
aux_icn,
solutions,
non_sltns,
dom_size,
eval(metric),
pop_size,
iter;
samples,
memoize,
parameters...
)
aux_results[i] = weights(aux_icn)
end
foreach(bv -> incsert!(results, bv), aux_results)
best = rand(findall(x -> x == maximum(results), results))
weights!(icn, best)
return best, results
end
struct ICNGeneticOptimizer <: ICNOptimizer
global_iter::Int
local_iter::Int
memoize::Bool
pop_size::Int
sampler::Union{Nothing, Function}
end
"""
ICNGeneticOptimizer(; kargs...)
Default constructor to learn an ICN through a Genetic Algorithm. Default `kargs` TBW.
"""
function ICNGeneticOptimizer(;
global_iter = Threads.nthreads(),
local_iter = 64,
memoize = false,
pop_size = 64,
sampler = nothing
)
return ICNGeneticOptimizer(global_iter, local_iter, memoize, pop_size, sampler)
end
"""
CompositionalNetworks.optimize!(icn, solutions, non_sltns, dom_size, metric, optimizer::ICNGeneticOptimizer; parameters...)
Extends the `optimize!` method to `ICNGeneticOptimizer`.
"""
function CompositionalNetworks.optimize!(
icn, solutions, non_sltns, dom_size, metric, optimizer::ICNGeneticOptimizer;
parameters...
)
return optimize!(
icn,
solutions,
non_sltns,
optimizer.global_iter,
optimizer.local_iter,
dom_size,
metric,
optimizer.pop_size;
optimizer.sampler,
optimizer.memoize,
parameters...
)
end
"""
ICNConfig(; metric = :hamming, optimizer = ICNGeneticOptimizer())
Constructor for `ICNConfig`. Defaults to hamming metric using a genetic algorithm.
"""
function ICNConfig(; metric = :hamming, optimizer = ICNGeneticOptimizer())
return ICNConfig(metric, optimizer)
end
@testitem "ICN: Genetic" tags=[:icn, :genetic] default_imports=false begin
using ConstraintDomains
using ConstraintLearning
using Test
domains = [domain([1, 2, 3, 4]) for i in 1:4]
compo = icn(domains, allunique)
@test compo([1, 2, 3, 3], dom_size = 4) > 0.0
end
| ConstraintLearning | https://github.com/JuliaConstraints/ConstraintLearning.jl.git |
|
[
"MIT"
] | 0.1.9 | fb00568e77176ce5a5e9266277b7a696cbc4e0f3 | code | 203 | """
const QUBOOptimizer = QUBOConstraints.AbstractOptimizer
An abstract type for optimizers used to learn QUBO matrices from constraints.
"""
const QUBOOptimizer = QUBOConstraints.AbstractOptimizer
| ConstraintLearning | https://github.com/JuliaConstraints/ConstraintLearning.jl.git |
|
[
"MIT"
] | 0.1.9 | fb00568e77176ce5a5e9266277b7a696cbc4e0f3 | code | 6153 | struct QUBOGradientOptimizer <: QUBOConstraints.AbstractOptimizer
binarization::Symbol
η::Float64
precision::Int
oversampling::Bool
end
"""
QUBOGradientOptimizer(; kargs...)
A QUBO optimizer based on gradient descent. Defaults TBW
"""
function QUBOGradientOptimizer(;
binarization = :one_hot,
η = 0.001,
precision = 5,
oversampling = false
)
return QUBOGradientOptimizer(binarization, η, precision, oversampling)
end
"""
predict(x, Q)
Return the predictions given by `Q` for a given configuration `x`.
"""
predict(x, Q) = transpose(x) * Q * x
"""
loss(x, y, Q)
Loss of the prediction given by `Q`, a training set `y`, and a given configuration `x`.
"""
loss(x, y, Q) = (predict(x, Q) .- y) .^ 2
"""
make_df(X, Q, penalty, binarization, domains)
DataFrame arrangement to output some basic evaluation of a matrix `Q`.
"""
function make_df(X, Q, penalty, binarization, domains)
df = DataFrame()
for (i, x) in enumerate(X)
if i == 1
df = DataFrame(transpose(x), :auto)
else
push!(df, transpose(x))
end
end
dim = length(df[1, :])
if binarization == :none
df[!, :penalty] = map(r -> penalty(Vector(r)), eachrow(df))
df[!, :predict] = map(r -> predict(Vector(r), Q), eachrow(df[:, 1:dim]))
else
df[!, :penalty] = map(
r -> penalty(binarize(Vector(r), domains; binarization)),
eachrow(df)
)
df[!, :predict] = map(
r -> predict(binarize(Vector(r), domains; binarization), Q),
eachrow(df[:, 1:dim])
)
end
min_false = minimum(
filter(:penalty => >(minimum(df[:, :penalty])), df)[:, :predict];
init = typemax(Int)
)
df[!, :shifted] = df[:, :predict] .- min_false
df[!, :accurate] = df[:, :penalty] .* df[:, :shifted] .≥ 0.0
return df
end
"""
preliminaries(args)
Preliminaries to the training process in a `QUBOGradientOptimizer` run.
"""
function preliminaries(X, domains, binarization)
if binarization == :none
n = length(first(X))
return X, zeros(n, n)
else
Y = map(x -> collect(binarize(x, domains; binarization)), X)
n = length(first(Y))
return Y, zeros(n, n)
end
end
function preliminaries(X, _)
n = length(first(X))
return X, zeros(n, n)
end
"""
train!(Q, X, penalty, η, precision, X_test, oversampling, binarization, domains)
Training inner method.
"""
function train!(Q, X, penalty, η, precision, X_test, oversampling, binarization, domains)
θ = params(Q)
try
penalty(first(X))
catch e
if isa(e, UndefKeywordError)
penalty = (x; dom_size = δ_extrema(Iterators.flatten(X))) -> penalty(
x; dom_size)
else
throw(e)
end
end
for x in (oversampling ? oversample(X, penalty) : X)
grads = gradient(() -> loss(x, penalty(x), Q), θ)
Q .-= η * grads[Q]
end
Q[:, :] = round.(precision * Q)
df = make_df(X_test, Q, penalty, binarization, domains)
return pretty_table(DataFrames.describe(df[
!, [:penalty, :predict, :shifted, :accurate]]))
end
"""
train(X, penalty[, d]; optimizer = QUBOGradientOptimizer(), X_test = X)
Learn a QUBO matrix on training set `X` for a constraint defined by `penalty` with optional domain information `d`. By default, it uses a `QUBOGradientOptimizer` and `X` as a testing set.
"""
function train(
X,
penalty,
domains::Vector{D};
optimizer = QUBOGradientOptimizer(),
X_test = X
) where {D <: DiscreteDomain}
Y, Q = preliminaries(X, domains, optimizer.binarization)
train!(
Q, Y, penalty, optimizer.η, optimizer.precision, X_test,
optimizer.oversampling, optimizer.binarization, domains
)
return Q
end
function train(
X,
penalty,
dom_stuff = nothing;
optimizer = QUBOGradientOptimizer(),
X_test = X
)
return train(X, penalty, to_domains(X, dom_stuff); optimizer, X_test)
end
## SECTION - Test Items
@testitem "QUBOConstraints" tags=[:qubo, :gradient] default_imports=false begin
using ConstraintLearning
using QUBOConstraints
X₃₃ = [rand(0:2, 3) for _ in 1:10]
X₃₃_test = [rand(0:2, 3) for _ in 1:100]
B₉ = [rand(Bool, 9) for _ in 1:10]
B₉_test = [rand(Bool, 9) for _ in 1:2000]
training_configs = [
Dict(
:info => "No binarization on ⟦0,2⟧³",
:train => X₃₃,
:test => X₃₃_test,
:encoding => :none,
:binarization => :none
),
Dict(
:info => "Domain Wall binarization on ⟦0,2⟧³",
:train => X₃₃,
:test => X₃₃_test,
:encoding => :none,
:binarization => :domain_wall
),
Dict(
:info => "One-Hot pre-encoded on ⟦0,2⟧³",
:train => B₉,
:test => B₉_test,
:encoding => :one_hot,
:binarization => :none
)
]
function all_different(x, encoding)
encoding == :none && (return allunique(x))
isv = if encoding == :one_hot
mapreduce(i -> is_valid(x[i:(i + 2)], Val(encoding)), *, 1:3:9)
else
mapreduce(i -> is_valid(x[i:(i + 1)], Val(encoding)), *, 1:2:6)
end
if isv
b = all_different(debinarize(x; binarization = encoding), :none)
return b ? -1.0 : 1.0
else
return length(x)
end
end
function all_different(x, encoding, binarization)
return all_different(x, encoding == :none ? binarization : encoding)
end
for config in training_configs
println("\nTest for $(config[:info])")
penalty = x -> all_different(x, config[:encoding], config[:binarization])
optimizer = QUBOGradientOptimizer(; binarization = config[:binarization])
qubo(config[:train], penalty; optimizer, X_test = config[:test])
# qubo(config[:train], penalty; optimizer, X_test = config[:test], icn_conf = ICNConfig())
end
end
| ConstraintLearning | https://github.com/JuliaConstraints/ConstraintLearning.jl.git |
|
[
"MIT"
] | 0.1.9 | fb00568e77176ce5a5e9266277b7a696cbc4e0f3 | code | 884 | @testset "Aqua.jl" begin
import Aqua
import ConstraintLearning
# TODO: Fix the broken tests and remove the `broken = true` flag
Aqua.test_all(
ConstraintLearning;
ambiguities = (broken = true,),
deps_compat = false,
piracies = (broken = false,),
unbound_args = (broken = false)
)
@testset "Ambiguities: ConstraintLearning" begin
# Aqua.test_ambiguities(ConstraintLearning;)
end
@testset "Piracies: ConstraintLearning" begin
# Aqua.test_piracies(ConstraintLearning;)
end
@testset "Dependencies compatibility (no extras)" begin
Aqua.test_deps_compat(
ConstraintLearning;
check_extras = false # ignore = [:Random]
)
end
@testset "Unbound type parameters" begin
# Aqua.test_unbound_args(ConstraintLearning;)
end
end
| ConstraintLearning | https://github.com/JuliaConstraints/ConstraintLearning.jl.git |
|
[
"MIT"
] | 0.1.9 | fb00568e77176ce5a5e9266277b7a696cbc4e0f3 | code | 59 | @testset "TestItemRunner" begin
@run_package_tests
end
| ConstraintLearning | https://github.com/JuliaConstraints/ConstraintLearning.jl.git |
|
[
"MIT"
] | 0.1.9 | fb00568e77176ce5a5e9266277b7a696cbc4e0f3 | code | 160 | using Test
using TestItemRunner
using TestItems
@testset "Package tests: ConstraintLearning" begin
include("Aqua.jl")
include("TestItemRunner.jl")
end
| ConstraintLearning | https://github.com/JuliaConstraints/ConstraintLearning.jl.git |
|
[
"MIT"
] | 0.1.9 | fb00568e77176ce5a5e9266277b7a696cbc4e0f3 | docs | 1221 | # ConstraintLearning
[](https://JuliaConstraints.github.io/ConstraintLearning.jl/stable)
[](https://JuliaConstraints.github.io/ConstraintLearning.jl/dev)
[](https://github.com/JuliaConstraints/ConstraintLearning.jl/actions/workflows/CI.yml?query=branch%3Amain)
[](https://codecov.io/gh/JuliaConstraints/ConstraintLearning.jl)
[](https://github.com/invenia/BlueStyle)
[](https://github.com/SciML/ColPrac)
[](https://JuliaCI.github.io/NanosoldierReports/pkgeval_badges/report.html)
## Citing
See [`CITATION.bib`](CITATION.bib) for the relevant reference(s).
| ConstraintLearning | https://github.com/JuliaConstraints/ConstraintLearning.jl.git |
|
[
"MIT"
] | 1.3.0 | 91d51d3b38c103a0e0820f2e4419812efdd7e7e5 | code | 1977 | using ModelingToolkit
using ModelingToolkitStandardLibrary.Electrical
using ModelingToolkitStandardLibrary.Blocks: Constant
using ModelingToolkitDesigner
using CairoMakie
using GLMakie
@component function PassThru2(; name)
@variables t
systems = @named begin
p1 = Pin()
p2 = Pin()
end
eqs = [connect(p1, p2)]
return ODESystem(eqs, t, [], []; name, systems)
end
@component function PassThru3(; name)
@variables t
systems = @named begin
p1 = Pin()
p2 = Pin()
p3 = Pin()
end
eqs = [connect(p1, p2, p3)]
return ODESystem(eqs, t, [], []; name, systems)
end
@component function Circuit(; name)
R = 1.0
C = 1.0
V = 1.0
@variables t
systems = @named begin
resistor = Resistor(R = R)
capacitor = Capacitor(C = C)
source = Voltage()
constant = Constant(k = V)
ground = Ground()
pt2 = PassThru2()
pt3 = PassThru3()
end
eqs = [
connect(resistor.p, pt2.p1)
connect(source.p, pt2.p2)
connect(source.n, pt3.p2)
connect(capacitor.n, pt3.p1)
connect(resistor.n, capacitor.p)
connect(ground.g, pt3.p3)
connect(source.V, constant.output)
]
# eqs = [connect(constant.output, source.V)
# connect(source.p, resistor.p)
# connect(resistor.n, capacitor.p)
# connect(capacitor.n, source.n, ground.g)]
ODESystem(eqs, t, [], []; systems, name)
end
@named rc = Circuit()
# using OrdinaryDiffEq
# sys = structural_simplify(rc)
# prob = ODEProblem(sys, Pair[], (0, 10.0))
# sol = solve(prob, Tsit5())
path = joinpath(@__DIR__, "design")
design = ODESystemDesign(rc, path)
GLMakie.set_theme!(Theme(; fontsize = 12))
ModelingToolkitDesigner.view(design)
# CairoMakie.set_theme!(Theme(;fontsize=12))
# fig = ModelingToolkitDesigner.view(design, false)
# save(joinpath(@__DIR__, "electrical.svg"), fig; resolution=(300,300))
| ModelingToolkitDesigner | https://github.com/bradcarman/ModelingToolkitDesigner.jl.git |
|
[
"MIT"
] | 1.3.0 | 91d51d3b38c103a0e0820f2e4419812efdd7e7e5 | code | 1190 | using ModelingToolkit
using ModelingToolkitDesigner
import ModelingToolkitStandardLibrary.Hydraulic.IsothermalCompressible as IC
import ModelingToolkitStandardLibrary.Blocks as B
@parameters t
@component function System(; name)
pars = []
systems = @named begin
fluid = IC.HydraulicFluid(; density = 876, bulk_modulus = 1.2e9, viscosity = 0.034)
stp = B.Step(; height = 10e5, start_time = 0.005)
src = IC.InputSource(; p_int = 0)
vol = IC.FixedVolume(; p_int = 0, vol = 10.0)
res = IC.Tube(5; p_int = 0, area = 0.01, length = 500.0)
end
eqs = [
connect(stp.output, src.p)
connect(src.port, res.port_a)
connect(vol.port, res.port_b)
connect(src.port, fluid)
]
ODESystem(eqs, t, [], pars; name, systems)
end
@named sys = System()
path = joinpath(@__DIR__, "design") # folder where visualization info is saved and retrieved
design = ODESystemDesign(sys, path);
ModelingToolkitDesigner.view(design)
# using CairoMakie
# CairoMakie.set_theme!(Theme(;fontsize=12))
# fig = ModelingToolkitDesigner.view(design, false)
# save(joinpath(@__DIR__, "hydraulic.svg"), fig; resolution=(400,200))
| ModelingToolkitDesigner | https://github.com/bradcarman/ModelingToolkitDesigner.jl.git |
|
[
"MIT"
] | 1.3.0 | 91d51d3b38c103a0e0820f2e4419812efdd7e7e5 | code | 1400 | using ModelingToolkit
using ModelingToolkitDesigner
using ModelingToolkitStandardLibrary.Blocks
import ModelingToolkitStandardLibrary.Mechanical.Translational as TV
@parameters t
D = Differential(t)
@component function PassThru3(; name)
@variables t
systems = @named begin
p1 = TV.MechanicalPort()
p2 = TV.MechanicalPort()
p3 = TV.MechanicalPort()
end
eqs = [connect(p1, p2, p3)]
return ODESystem(eqs, t, [], []; name, systems)
end
@component function MassSpringDamper(; name)
systems = @named begin
dv = TV.Damper(d = 1, v_a_0 = 1)
sv = TV.Spring(k = 1, v_a_0 = 1, delta_s_0 = 1)
bv = TV.Mass(m = 1, v_0 = 1)
gv = TV.Fixed()
pt1 = PassThru3()
pt2 = PassThru3()
end
eqs = [
connect(bv.flange, pt2.p2)
connect(sv.flange_a, pt2.p1)
connect(dv.flange_a, pt2.p3)
connect(dv.flange_b, pt1.p3)
connect(sv.flange_b, pt1.p1)
connect(gv.flange, pt1.p2)
]
return ODESystem(eqs, t, [], []; name, systems)
end
@named msd = MassSpringDamper()
path = joinpath(@__DIR__, "design")
design = ODESystemDesign(msd, path)
ModelingToolkitDesigner.view(design)
# using CairoMakie
# CairoMakie.set_theme!(Theme(;fontsize=12))
# fig = ModelingToolkitDesigner.view(design, false)
# save(joinpath(@__DIR__, "mechanical.svg"), fig; resolution=(400,200))
| ModelingToolkitDesigner | https://github.com/bradcarman/ModelingToolkitDesigner.jl.git |
|
[
"MIT"
] | 1.3.0 | 91d51d3b38c103a0e0820f2e4419812efdd7e7e5 | code | 203 | connect(resistor.p, pt2.p1)
connect(source.p, pt2.p2)
connect(source.n, pt3.p2)
connect(capacitor.n, pt3.p1)
connect(resistor.n, capacitor.p)
connect(ground.g, pt3.p3)
connect(source.V, constant.output)
| ModelingToolkitDesigner | https://github.com/bradcarman/ModelingToolkitDesigner.jl.git |
|
[
"MIT"
] | 1.3.0 | 91d51d3b38c103a0e0820f2e4419812efdd7e7e5 | code | 170 | connect(bv.flange, pt2.p2)
connect(sv.flange_a, pt2.p1)
connect(dv.flange_a, pt2.p3)
connect(dv.flange_b, pt1.p3)
connect(sv.flange_b, pt1.p1)
connect(gv.flange, pt1.p2)
| ModelingToolkitDesigner | https://github.com/bradcarman/ModelingToolkitDesigner.jl.git |
|
[
"MIT"
] | 1.3.0 | 91d51d3b38c103a0e0820f2e4419812efdd7e7e5 | code | 112 | connect(stp.output, src.p)
connect(src.port, res.port_a)
connect(vol.port, res.port_b)
connect(src.port, fluid)
| ModelingToolkitDesigner | https://github.com/bradcarman/ModelingToolkitDesigner.jl.git |
|
[
"MIT"
] | 1.3.0 | 91d51d3b38c103a0e0820f2e4419812efdd7e7e5 | code | 38992 | module ModelingToolkitDesigner
using GLMakie
using CairoMakie
using ModelingToolkit
using FilterHelpers
using FileIO
using TOML
using ModelingToolkitStandardLibrary.Blocks: AnalysisPoint
#TODO: ctrl + up/down/left/right gives bigger jumps
const Δh = 0.05
const dragging = Ref(false)
abstract type DesignMap end
struct DesignColorMap <: DesignMap
domain::String
color::Symbol
end
const design_colors = DesignMap[
DesignColorMap("ModelingToolkitStandardLibrary.Electrical.Pin", :tomato)
DesignColorMap(
"ModelingToolkitStandardLibrary.Mechanical.Translational.MechanicalPort",
:yellowgreen,
)
DesignColorMap(
"ModelingToolkitStandardLibrary.Mechanical.TranslationalPosition.Flange",
:green,
)
DesignColorMap(
"ModelingToolkitStandardLibrary.Mechanical.TranslationalPosition.Support",
:green,
)
DesignColorMap("ModelingToolkitStandardLibrary.Mechanical.Rotational.Flange", :green)
DesignColorMap("ModelingToolkitStandardLibrary.Mechanical.Rotational.Support", :green)
DesignColorMap("ModelingToolkitStandardLibrary.Thermal.HeatPort", :red)
DesignColorMap(
"ModelingToolkitStandardLibrary.Magnetic.FluxTubes.MagneticPort",
:purple,
)
DesignColorMap(
"ModelingToolkitStandardLibrary.Hydraulic.IsothermalCompressible.HydraulicPort",
:blue,
)
DesignColorMap(
"ModelingToolkitStandardLibrary.Hydraulic.IsothermalCompressible.HydraulicFluid",
:blue,
)
]
function add_color(system::ODESystem, color::Symbol)
@assert ModelingToolkit.isconnector(system) "The `system` must be a connector"
type = string(system.gui_metadata.type)
map = DesignColorMap(type, color)
push!(design_colors, map)
println("Added $map to `ModelingToolkitDesigner.design_colors`")
end
mutable struct ODESystemDesign
# parameters::Vector{Parameter}
# states::Vector{State}
parent::Union{Symbol,Nothing}
system::ODESystem
system_color::Symbol
components::Vector{ODESystemDesign}
connectors::Vector{ODESystemDesign}
connections::Vector{Tuple{ODESystemDesign,ODESystemDesign}}
xy::Observable{Tuple{Float64,Float64}}
icon::Union{String,Nothing}
color::Observable{Symbol}
wall::Observable{Symbol}
file::String
end
Base.show(io::IO, x::ODESystemDesign) = print(io, x.system.name)
Base.show(io::IO, x::Tuple{ODESystemDesign,ODESystemDesign}) = print(
io,
"$(x[1].parent).$(x[1].system.name) $(round.(x[1].xy[]; digits=2)) <---> $(x[2].parent).$(x[2].system.name) $(round.(x[2].xy[]; digits=2))",
)
Base.copy(x::Tuple{ODESystemDesign,ODESystemDesign}) = (copy(x[1]), copy(x[2]))
Base.copy(x::ODESystemDesign) = ODESystemDesign(
x.parent,
x.system,
x.system_color,
copy.(x.components),
copy.(x.connectors),
copy.(x.connections),
Observable(x.xy[]),
x.icon,
Observable(x.system_color),
Observable(x.wall[]),
x.file,
)
function ODESystemDesign(
system::ODESystem,
design_path::String;
x = 0.0,
y = 0.0,
icon = nothing,
wall = :E,
parent = nothing,
kwargs...,
)
file = design_file(system, design_path)
design_dict = if isfile(file)
TOML.parsefile(file)
else
Dict()
end
systems = filter(x -> typeof(x) == ODESystem, ModelingToolkit.get_systems(system))
components = ODESystemDesign[]
connectors = ODESystemDesign[]
connections = Tuple{ODESystemDesign,ODESystemDesign}[]
if !isempty(systems)
process_children!(
components,
systems,
design_dict,
design_path,
system.name,
false;
kwargs...,
)
process_children!(
connectors,
systems,
design_dict,
design_path,
system.name,
true;
kwargs...,
)
for wall in [:E, :W, :N, :S]
connectors_on_wall = filter(x -> get_wall(x) == wall, connectors)
n = length(connectors_on_wall)
if n > 1
i = 0
for conn in connectors_on_wall
order = get_wall_order(conn)
i = max(i, order) + 1
if order == 0
conn.wall[] = Symbol(wall, i)
end
end
end
end
for eq in equations(system)
if (eq.rhs isa Connection) && isnothing(eq.lhs.systems) #filters out domain_connect #TODO: handle case of domain_connect connection with fluid
conns = []
for connector in eq.rhs.systems
if contains(string(connector.name), '₊')
# connector of child system
conn_parent, child = split(string(connector.name), '₊')
child_design = filtersingle(
x -> string(x.system.name) == conn_parent,
components,
)
if !isnothing(child_design)
connector_design = filtersingle(
x -> string(x.system.name) == child,
child_design.connectors,
)
else
connector_design = nothing
end
else
# connector of parent system
connector_design =
filtersingle(x -> x.system.name == connector.name, connectors)
end
if !isnothing(connector_design)
push!(conns, connector_design)
end
end
for i = 2:length(conns)
push!(connections, (conns[i-1], conns[i]))
end
end
if eq.rhs isa AnalysisPoint
conns = []
for connector in [eq.rhs.in, eq.rhs.out]
if contains(string(connector.name), '₊')
# connector of child system
conn_parent, child = split(string(connector.name), '₊')
child_design = filtersingle(
x -> string(x.system.name) == conn_parent,
components,
)
if !isnothing(child_design)
connector_design = filtersingle(
x -> string(x.system.name) == child,
child_design.connectors,
)
else
connector_design = nothing
end
else
# connector of parent system
connector_design =
filtersingle(x -> x.system.name == connector.name, connectors)
end
if !isnothing(connector_design)
push!(conns, connector_design)
end
end
for i = 2:length(conns)
push!(connections, (conns[i-1], conns[i]))
end
end
end
end
xy = Observable((x, y))
color = get_color(system)
if wall isa String
wall = Symbol(wall)
end
return ODESystemDesign(
parent,
system,
color,
components,
connectors,
connections,
xy,
icon,
Observable(color),
Observable(wall),
file,
)
end
is_pass_thru(design::ODESystemDesign) = is_pass_thru(design.system)
function is_pass_thru(system::ODESystem)
types = split(string(system.gui_metadata.type), '.')
component_type = types[end]
return startswith(component_type, "PassThru")
end
function design_file(system::ODESystem, path::String)
@assert !isnothing(system.gui_metadata) "ODESystem must use @component: $(system.name)"
# path = joinpath(@__DIR__, "designs")
if !isdir(path)
mkdir(path)
end
parts = split(string(system.gui_metadata.type), '.')
for part in parts[1:end-1]
path = joinpath(path, part)
if !isdir(path)
mkdir(path)
end
end
file = joinpath(path, "$(parts[end]).toml")
return file
end
function process_children!(
children::Vector{ODESystemDesign},
systems::Vector{ODESystem},
design_dict::Dict,
design_path::String,
parent::Symbol,
is_connector = false;
connectors...,
)
systems = filter(x -> ModelingToolkit.isconnector(x) == is_connector, systems)
if !isempty(systems)
for (i, system) in enumerate(systems)
# key = Symbol(namespace, "₊", system.name)
key = string(system.name)
kwargs = if haskey(design_dict, key)
design_dict[key]
else
Dict()
end
kwargs_pair = Pair[]
push!(kwargs_pair, :parent => parent)
if !is_connector
#if x and y are missing, add defaults
if !haskey(kwargs, "x") & !haskey(kwargs, "y")
push!(kwargs_pair, :x => i * 3 * Δh)
push!(kwargs_pair, :y => i * Δh)
end
# r => wall for icon rotation
if haskey(kwargs, "r")
push!(kwargs_pair, :wall => kwargs["r"])
end
else
if haskey(connectors, safe_connector_name(system.name))
push!(kwargs_pair, :wall => connectors[safe_connector_name(system.name)])
end
end
for (key, value) in kwargs
push!(kwargs_pair, Symbol(key) => value)
end
push!(kwargs_pair, :icon => find_icon(system, design_path))
push!(
children,
ODESystemDesign(system, design_path; NamedTuple(kwargs_pair)...),
)
end
end
end
function get_design_path(design::ODESystemDesign)
type = string(design.system.gui_metadata.type)
parts = split(type, '.')
path = joinpath(parts[1:end-1]..., "$(parts[end]).toml")
return replace(design.file, path => "")
end
function get_icons(system::ODESystem, design_path::String)
type = string(system.gui_metadata.type)
path = split(type, '.')
pkg_location = Base.find_package(string(path[1]))
bases = if !isnothing(pkg_location)
[
design_path
joinpath(pkg_location, "..", "..", "icons")
joinpath(@__DIR__, "..", "icons")
]
else
[
design_path
joinpath(@__DIR__, "..", "icons")
]
end
icons = [joinpath(base, path[1:end-1]..., "$(path[end]).png") for base in bases]
return abspath.(icons)
end
find_icon(design::ODESystemDesign) = find_icon(design.system, get_design_path(design))
function find_icon(system::ODESystem, design_path::String)
icons = get_icons(system, design_path)
for icon in icons
isfile(icon) && return icon
end
return joinpath(@__DIR__, "..", "icons", "NotFound.png")
end
function is_tuple_approx(a::Tuple{<:Number,<:Number}, b::Tuple{<:Number,<:Number}; atol)
r1 = isapprox(a[1], b[1]; atol)
r2 = isapprox(a[2], b[2]; atol)
return all([r1, r2])
end
get_change(::Val) = (0.0, 0.0)
get_change(::Val{Keyboard.up}) = (0.0, +Δh / 5)
get_change(::Val{Keyboard.down}) = (0.0, -Δh / 5)
get_change(::Val{Keyboard.left}) = (-Δh / 5, 0.0)
get_change(::Val{Keyboard.right}) = (+Δh / 5, 0.0)
function next_wall(current_wall)
if current_wall == :N
:E
elseif current_wall == :W
:N
elseif current_wall == :S
:W
elseif current_wall == :E
:S
end
end
function view(design::ODESystemDesign, interactive = true)
if interactive
GLMakie.activate!(inline=false)
else
CairoMakie.activate!()
end
fig = Figure()
title = if isnothing(design.parent)
"$(design.system.name) [$(design.file)]"
else
"$(design.parent).$(design.system.name) [$(design.file)]"
end
ax = Axis(
fig[2:11, 1:10];
aspect = DataAspect(),
yticksvisible = false,
xticksvisible = false,
yticklabelsvisible = false,
xticklabelsvisible = false,
xgridvisible = false,
ygridvisible = false,
bottomspinecolor = :transparent,
leftspinecolor = :transparent,
rightspinecolor = :transparent,
topspinecolor = :transparent,
)
if interactive
connect_button = Button(fig[12, 1]; label = "connect", fontsize = 12)
clear_selection_button =
Button(fig[12, 2]; label = "clear selection", fontsize = 12)
next_wall_button = Button(fig[12, 3]; label = "move node", fontsize = 12)
align_horrizontal_button = Button(fig[12, 4]; label = "align horz.", fontsize = 12)
align_vertical_button = Button(fig[12, 5]; label = "align vert.", fontsize = 12)
open_button = Button(fig[12, 6]; label = "open", fontsize = 12)
rotate_button = Button(fig[12, 7]; label = "rotate", fontsize=12)
mode_toggle = Toggle(fig[12, 8])
save_button = Button(fig[12, 10]; label = "save", fontsize = 12)
Label(fig[1, :], title; halign = :left, fontsize = 11)
Label(fig[2,:], "keyboard: m - toggle mouse dragging, c - connect, r - rotate"; halign = :left, fontsize=10)
end
for component in design.components
add_component!(ax, component)
for connector in component.connectors
notify(connector.wall)
end
notify(component.xy)
end
for connector in design.connectors
add_component!(ax, connector)
notify(connector.xy)
end
for connection in design.connections
connect!(ax, connection)
end
if interactive
on(events(fig).mousebutton, priority = 2) do event
if event.button == Mouse.left
if event.action == Mouse.press
# if Keyboard.s in events(fig).keyboardstate
# Delete marker
plt, i = pick(fig)
if !isnothing(plt)
if plt isa Image
image = plt
xobservable = image[1]
xvalues = xobservable[]
yobservable = image[2]
yvalues = yobservable[]
x = xvalues.left + Δh * 0.8
y = yvalues.left + Δh * 0.8
selected_system = filtersingle(
s -> is_tuple_approx(s.xy[], (x, y); atol = 1e-3),
[design.components; design.connectors],
)
if isnothing(selected_system)
x = xvalues.left + Δh * 0.8 * 0.5
y = yvalues.left + Δh * 0.8 * 0.5
selected_system = filterfirst(
s -> is_tuple_approx(s.xy[], (x, y); atol = 1e-3),
[design.components; design.connectors],
)
if isnothing(selected_system)
@warn "clicked an image at ($(round(x; digits=1)), $(round(y; digits=1))), but no system design found!"
else
selected_system.color[] = :pink
dragging[] = true
end
else
selected_system.color[] = :pink
dragging[] = true
end
elseif plt isa Scatter
point = plt
observable = point[1]
values = observable[]
geometry_point = Float64.(values[1])
x = geometry_point[1]
y = geometry_point[2]
selected_component = filtersingle(
c -> is_tuple_approx(c.xy[], (x, y); atol = 1e-3),
design.components,
)
if !isnothing(selected_component)
selected_component.color[] = :pink
else
all_connectors =
vcat([s.connectors for s in design.components]...)
selected_connector = filtersingle(
c -> is_tuple_approx(c.xy[], (x, y); atol = 1e-3),
all_connectors,
)
if !isnothing(selected_connector)
selected_connector.color[] = :pink
end
end
elseif plt isa Mesh
triangles = plt[1][]
points = map(x->sum(x.points)/length(x.points), triangles)
x,y = sum(points)/length(points)
selected_component = filtersingle(
c -> is_tuple_approx(c.xy[], (x, y); atol = 1e-3),
design.components,
)
if !isnothing(selected_component)
selected_component.color[] = :pink
dragging[] = true
end
end
end
Consume(true)
elseif event.action == Mouse.release
dragging[] = false
Consume(true)
end
end
if event.button == Mouse.right
clear_selection(design)
Consume(true)
end
return Consume(false)
end
on(events(fig).mouseposition, priority = 2) do mp
if dragging[]
for sub_design in [design.components; design.connectors]
if sub_design.color[] == :pink
position = mouseposition(ax)
sub_design.xy[] = (position[1], position[2])
break #only move one system for mouse drag
end
end
return Consume(true)
end
return Consume(false)
end
on(events(fig).keyboardbutton) do event
if event.action == Keyboard.press
if event.key == Keyboard.m
dragging[] = !dragging[]
elseif event.key == Keyboard.c
connect!(ax, design)
elseif event.key == Keyboard.r
rotate(design)
else
change = get_change(Val(event.key))
if change != (0.0, 0.0)
for sub_design in [design.components; design.connectors]
if sub_design.color[] == :pink
xc = sub_design.xy[][1]
yc = sub_design.xy[][2]
sub_design.xy[] = (xc + change[1], yc + change[2])
end
end
reset_limits!(ax)
return Consume(true)
end
end
end
end
on(connect_button.clicks) do clicks
connect!(ax, design)
end
on(clear_selection_button.clicks) do clicks
clear_selection(design)
end
#TODO: fix the ordering too
on(next_wall_button.clicks) do clicks
for component in design.components
for connector in component.connectors
if connector.color[] == :pink
current_wall = get_wall(connector)
current_order = get_wall_order(connector)
if current_order > 1
connectors_on_wall = filter(x -> get_wall(x) == current_wall, component.connectors)
for cow in connectors_on_wall
order = max(get_wall_order(cow), 1)
if order == current_order - 1
cow.wall[] = Symbol(current_wall, current_order)
end
if order == current_order
cow.wall[] = Symbol(current_wall, current_order - 1)
end
end
else
connectors_on_wall = filter(x -> get_wall(x) == next_wall(current_wall), component.connectors)
# connector is added to wall, need to fix any un-ordered connectors
for cow in connectors_on_wall
order = get_wall_order(cow)
if order == 0
cow.wall[] = Symbol(next_wall(current_wall), 1)
end
end
current_order = length(connectors_on_wall) + 1
if current_order > 1
connector.wall[] = Symbol(next_wall(current_wall), current_order)
else
connector.wall[] = next_wall(current_wall)
end
# connector is leaving wall, need to reduce the order
connectors_on_wall = filter(x -> get_wall(x) == current_wall, component.connectors)
if length(connectors_on_wall) > 1
for cow in connectors_on_wall
order = get_wall_order(cow)
if order == 0
cow.wall[] = Symbol(current_wall, 1)
else
cow.wall[] = Symbol(current_wall, order - 1)
end
end
else
for cow in connectors_on_wall
cow.wall[] = current_wall
end
end
end
end
end
end
end
on(align_horrizontal_button.clicks) do clicks
align(design, :horrizontal)
end
on(align_vertical_button.clicks) do clicks
align(design, :vertical)
end
on(open_button.clicks) do clicks
for component in design.components
if component.color[] == :pink
view_design =
ODESystemDesign(component.system, get_design_path(component))
view_design.parent = design.system.name
fig_ = view(view_design)
display(GLMakie.Screen(), fig_)
break
end
end
end
on(save_button.clicks) do clicks
save_design(design)
end
on(mode_toggle.active) do val
toggle_pass_thrus(design, val)
end
on(rotate_button.clicks) do clicks
rotate(design)
end
end
toggle_pass_thrus(design, !interactive)
return fig
end
function toggle_pass_thrus(design::ODESystemDesign, hide::Bool)
for component in design.components
if is_pass_thru(component)
if hide
component.color[] = :transparent
for connector in component.connectors
connector.color[] = :transparent
end
else
component.color[] = component.system_color
for connector in component.connectors
connector.color[] = connector.system_color
end
end
else
end
end
end
function rotate(design::ODESystemDesign)
for sub_design in [design.components; design.connectors]
if sub_design.color[] == :pink
sub_design.wall[] = next_wall(get_wall(sub_design))
end
end
end
function align(design::ODESystemDesign, type)
xs = Float64[]
ys = Float64[]
for sub_design in [design.components; design.connectors]
if sub_design.color[] == :pink
x, y = sub_design.xy[]
push!(xs, x)
push!(ys, y)
end
end
ym = sum(ys) / length(ys)
xm = sum(xs) / length(xs)
for sub_design in [design.components; design.connectors]
if sub_design.color[] == :pink
x, y = sub_design.xy[]
if type == :horrizontal
sub_design.xy[] = (x, ym)
elseif type == :vertical
sub_design.xy[] = (xm, y)
end
end
end
end
function clear_selection(design::ODESystemDesign)
for component in design.components
for connector in component.connectors
connector.color[] = connector.system_color
end
component.color[] = :black
end
for connector in design.connectors
connector.color[] = connector.system_color
end
end
function connect!(ax::Axis, design::ODESystemDesign)
all_connectors = vcat([s.connectors for s in design.components]...)
push!(all_connectors, design.connectors...)
selected_connectors = ODESystemDesign[]
for connector in all_connectors
if connector.color[] == :pink
push!(selected_connectors, connector)
connector.color[] = connector.system_color
end
end
if length(selected_connectors) > 1
connect!(ax, (selected_connectors[1], selected_connectors[2]))
push!(design.connections, (selected_connectors[1], selected_connectors[2]))
end
end
function connect!(ax::Axis, connection::Tuple{ODESystemDesign,ODESystemDesign})
xs = Observable(Float64[])
ys = Observable(Float64[])
update = () -> begin
empty!(xs[])
empty!(ys[])
for connector in connection
push!(xs[], connector.xy[][1])
push!(ys[], connector.xy[][2])
end
notify(xs)
notify(ys)
end
style = :solid
for connector in connection
s = get_style(connector)
if s != :solid
style = s
end
on(connector.xy) do val
update()
end
end
update()
lines!(ax, xs, ys; color = connection[1].color[], linestyle = style)
end
get_text_alignment(wall::Symbol) = get_text_alignment(Val(wall))
get_text_alignment(::Val{:E}) = (:left, :top)
get_text_alignment(::Val{:W}) = (:right, :top)
get_text_alignment(::Val{:S}) = (:left, :top)
get_text_alignment(::Val{:N}) = (:left, :bottom)
get_color(design::ODESystemDesign) = get_color(design.system)
function get_color(system::ODESystem)
if !isnothing(system.gui_metadata)
domain = string(system.gui_metadata.type)
# domain_parts = split(full_domain, '.')
# domain = join(domain_parts[1:end-1], '.')
map = filtersingle(
x -> (x.domain == domain) & (typeof(x) == DesignColorMap),
design_colors,
)
if !isnothing(map)
return map.color
end
end
return :black
end
get_style(design::ODESystemDesign) = get_style(design.system)
function get_style(system::ODESystem)
sts = ModelingToolkit.get_unknowns(system)
if length(sts) == 1
s = first(sts)
vtype = ModelingToolkit.get_connection_type(s)
if vtype === ModelingToolkit.Flow
return :dash
end
end
return :solid
end
function draw_box!(ax::Axis, design::ODESystemDesign)
xo = Observable(zeros(5))
yo = Observable(zeros(5))
points = Observable([(0., 0.), (1., 0.), (1., 1.), (0., 1.)])
Δh_, linewidth = if ModelingToolkit.isconnector(design.system)
0.6 * Δh, 2
else
Δh, 1
end
on(design.xy) do val
x = val[1]
y = val[2]
xo[], yo[] = box(x, y, Δh_)
new_points = Tuple{Float64, Float64}[]
for i=1:4
push!(new_points, (xo[][i], yo[][i]))
end
points[] = new_points
end
poly!(ax, points, color=:white)
lines!(ax, xo, yo; color = design.color, linewidth)
if !isempty(design.components)
xo2 = Observable(zeros(5))
yo2 = Observable(zeros(5))
on(design.xy) do val
x = val[1]
y = val[2]
xo2[], yo2[] = box(x, y, Δh_ * 1.1)
end
lines!(ax, xo2, yo2; color = design.color, linewidth)
end
end
function draw_passthru!(ax::Axis, design::ODESystemDesign)
xo = Observable(0.0)
yo = Observable(0.0)
on(design.xy) do val
x = val[1]
y = val[2]
xo[] = x
yo[] = y
end
scatter!(ax, xo, yo; color = first(design.connectors).system_color, marker = :circle)
for connector in design.connectors
cxo = Observable(zeros(2))
cyo = Observable(zeros(2))
function update()
cxo[] = [connector.xy[][1], design.xy[][1]]
cyo[] = [connector.xy[][2], design.xy[][2]]
end
on(connector.xy) do val
update()
end
on(design.xy) do val
update()
end
lines!(ax, cxo, cyo; color = connector.system_color)
end
end
function draw_icon!(ax::Axis, design::ODESystemDesign)
xo = Observable((0.0,0.0))
yo = Observable((0.0,0.0))
scale = if ModelingToolkit.isconnector(design.system)
0.5 * 0.8
else
0.8
end
on(design.xy) do val
x = val[1]
y = val[2]
xo[] = (x - Δh * scale, x + Δh * scale)
yo[] = (y - Δh * scale, y + Δh * scale)
end
if !isnothing(design.icon)
imgd = Observable(load(design.icon))
update() = begin
img = load(design.icon)
w = get_wall(design)
imgd[] = if w == :E
rotr90(img)
elseif w == :S
rotr90(rotr90(img))
elseif w == :W
rotr90(rotr90(rotr90(img)))
elseif w == :N
img
end
end
update()
on(design.wall) do val
update()
end
image!(ax, xo, yo, imgd)
end
end
function draw_label!(ax::Axis, design::ODESystemDesign)
xo = Observable(0.0)
yo = Observable(0.0)
scale = if ModelingToolkit.isconnector(design.system)
1 + 0.75 * 0.5
else
0.925
end
on(design.xy) do val
x = val[1]
y = val[2]
xo[] = x
yo[] = y - Δh * scale
end
text!(ax, xo, yo; text = string(design.system.name), align = (:center, :bottom))
end
function draw_nodes!(ax::Axis, design::ODESystemDesign)
xo = Observable(0.0)
yo = Observable(0.0)
on(design.xy) do val
x = val[1]
y = val[2]
xo[] = x
yo[] = y
end
update =
(connector) -> begin
connectors_on_wall =
filter(x -> get_wall(x) == get_wall(connector), design.connectors)
n_items = length(connectors_on_wall)
delta = 2 * Δh / (n_items + 1)
sort!(connectors_on_wall, by=x->x.wall[])
for i = 1:n_items
x, y = get_node_position(get_wall(connector), delta, i)
connectors_on_wall[i].xy[] = (x + xo[], y + yo[])
end
end
for connector in design.connectors
on(connector.wall) do val
update(connector)
end
on(design.xy) do val
update(connector)
end
draw_node!(ax, connector)
draw_node_label!(ax, connector)
end
end
function draw_node!(ax::Axis, connector::ODESystemDesign)
xo = Observable(0.0)
yo = Observable(0.0)
on(connector.xy) do val
x = val[1]
y = val[2]
xo[] = x
yo[] = y
end
scatter!(ax, xo, yo; marker = :rect, color = connector.color, markersize = 15)
end
function draw_node_label!(ax::Axis, connector::ODESystemDesign)
xo = Observable(0.0)
yo = Observable(0.0)
alignment = Observable((:left, :top))
on(connector.xy) do val
x = val[1]
y = val[2]
xt, yt = get_node_label_position(get_wall(connector), x, y)
xo[] = xt
yo[] = yt
alignment[] = get_text_alignment(get_wall(connector))
end
scene = GLMakie.Makie.parent_scene(ax)
current_font_size = theme(scene, :fontsize)
text!(
ax,
xo,
yo;
text = string(connector.system.name),
color = connector.color,
align = alignment,
fontsize = current_font_size[] * 0.625,
)
end
get_wall(design::ODESystemDesign) = Symbol(string(design.wall[])[1])
function get_wall_order(design::ODESystemDesign)
wall = string(design.wall[])
if length(wall) > 1
order = tryparse(Int, wall[2:end])
if isnothing(order)
order = 1
end
return order
else
return 0
end
end
get_node_position(w::Symbol, delta, i) = get_node_position(Val(w), delta, i)
get_node_label_position(w::Symbol, x, y) = get_node_label_position(Val(w), x, y)
get_node_position(::Val{:N}, delta, i) = (delta * i - Δh, +Δh)
get_node_label_position(::Val{:N}, x, y) = (x + Δh / 10, y + Δh / 5)
get_node_position(::Val{:S}, delta, i) = (delta * i - Δh, -Δh)
get_node_label_position(::Val{:S}, x, y) = (x + Δh / 10, y - Δh / 5)
get_node_position(::Val{:E}, delta, i) = (+Δh, delta * i - Δh)
get_node_label_position(::Val{:E}, x, y) = (x + Δh / 5, y)
get_node_position(::Val{:W}, delta, i) = (-Δh, delta * i - Δh)
get_node_label_position(::Val{:W}, x, y) = (x - Δh / 5, y)
function add_component!(ax::Axis, design::ODESystemDesign)
draw_box!(ax, design)
draw_nodes!(ax, design)
if is_pass_thru(design)
draw_passthru!(ax, design)
else
draw_icon!(ax, design)
draw_label!(ax, design)
end
end
# box(model::ODESystemDesign, Δh = 0.05) = box(model.xy[][1], model.xy[],[2] Δh)
function box(x, y, Δh = 0.05)
xs = [x + Δh, x - Δh, x - Δh, x + Δh, x + Δh]
ys = [y + Δh, y + Δh, y - Δh, y - Δh, y + Δh]
return xs, ys
end
# function get_design_code(design::ODESystemDesign)
# println("[")
# for child_design in design.systems
# println("\t$(design.system.name).$(child_design.system.name) => (x=$(round(child_design.xy[][1]; digits=2)), y=$(round(child_design.xy[][2]; digits=2)))")
# for node in child_design.connectors
# if node.wall[] != :E
# println("\t$(design.system.name).$(child_design.system.name).$(node.system.name) => (wall=:$(node.wall[]),)")
# end
# end
# end
# println()
# for node in design.connectors
# println("\t$(design.system.name).$(node.system.name) => (x=$(round(node.xy[][1]; digits=2)), y=$(round(node.xy[][2]; digits=2)))")
# end
# println("]")
# end
function connection_part(design::ODESystemDesign, connection::ODESystemDesign)
if connection.parent == design.system.name
return "$(connection.system.name)"
else
return "$(connection.parent).$(connection.system.name)"
end
end
connection_code(design::ODESystemDesign) = connection_code(stdout, design)
function connection_code(io::IO, design::ODESystemDesign)
for connection in design.connections
println(
io,
"connect($(connection_part(design, connection[1])), $(connection_part(design, connection[2])))",
)
end
end
safe_connector_name(name::Symbol) = Symbol("_$name")
function save_design(design::ODESystemDesign)
design_dict = Dict()
for component in design.components
x, y = component.xy[]
pairs = Pair{Symbol,Any}[
:x => round(x; digits = 2)
:y => round(y; digits = 2)
]
if component.wall[] != :E
push!(pairs,
:r => string(component.wall[])
)
end
for connector in component.connectors
if connector.wall[] != :E #don't use get_wall() here, need to preserve E1, E2, etc
push!(pairs, safe_connector_name(connector.system.name) => string(connector.wall[]))
end
end
design_dict[component.system.name] = Dict(pairs)
end
for connector in design.connectors
x, y = connector.xy[]
pairs = Pair{Symbol,Any}[
:x => round(x; digits = 2)
:y => round(y; digits = 2)
]
design_dict[connector.system.name] = Dict(pairs)
end
save_design(design_dict, design.file)
connection_file = replace(design.file, ".toml" => ".jl")
open(connection_file, "w") do io
connection_code(io, design)
end
end
function save_design(design_dict::Dict, file::String)
open(file, "w") do io
TOML.print(io, design_dict; sorted = true) do val
if val isa Symbol
return string(val)
end
end
end
end
# macro input(file)
# s = if isfile(file)
# read(file, String)
# else
# ""
# end
# e = Meta.parse(s)
# esc(e)
# end
export ODESystemDesign, DesignColorMap, connection_code, add_color
end # module ModelingToolkitDesigner
| ModelingToolkitDesigner | https://github.com/bradcarman/ModelingToolkitDesigner.jl.git |
|
[
"MIT"
] | 1.3.0 | 91d51d3b38c103a0e0820f2e4419812efdd7e7e5 | code | 2129 | using ModelingToolkitDesigner
using ModelingToolkit, Test
import ModelingToolkitStandardLibrary.Hydraulic.IsothermalCompressible as IC
import ModelingToolkitStandardLibrary.Blocks as B
import ModelingToolkitStandardLibrary.Mechanical.Translational as T
using ModelingToolkitDesigner: ODESystemDesign, DesignColorMap, DesignMap
@parameters t
D = Differential(t)
@component function system(N; name)
pars = []
systems = @named begin
fluid = IC.HydraulicFluid()
stp = B.Step(;
height = 10e5,
offset = 0,
start_time = 0.005,
duration = Inf,
smooth = 0,
)
src = IC.Pressure(; p_int = 0)
vol = IC.FixedVolume(; p_int = 0, vol = 10.0)
res = IC.Tube(N; p_int = 0, area = 0.01, length = 500.0)
end
eqs = Equation[
connect(stp.output, src.p)
connect(src.port, res.port_a)
connect(vol.port, res.port_b)
connect(src.port, fluid)
]
ODESystem(eqs, t, [], pars; name, systems)
end
@named sys = system(5)
@test ModelingToolkitDesigner.get_color(sys.vol.port) == :blue
@test ModelingToolkitDesigner.get_color(sys.vol) == :black
path = joinpath(@__DIR__, "designs");
fixed_volume_icon_ans =
raw"icons\ModelingToolkitStandardLibrary\Hydraulic\IsothermalCompressible\FixedVolume.png"
fixed_volume_icon_ans = abspath(fixed_volume_icon_ans)
fixed_volume_icon_ans = normpath(fixed_volume_icon_ans)
fixed_volume_icon_ans = lowercase(fixed_volume_icon_ans)
fixed_volume_icon = ModelingToolkitDesigner.find_icon(sys.vol, path)
fixed_volume_icon = abspath(fixed_volume_icon)
fixed_volume_icon = normpath(fixed_volume_icon)
fixed_volume_icon = lowercase(fixed_volume_icon)
#TODO: How can I make this work? normpath is not doing what I think it should
# @test fixed_volume_icon == fixed_volume_icon_ans
design = ODESystemDesign(sys, path);
@test design.components[2].xy[] == (0.58, 0.0)
@test lowercase(abspath(design.components[3].icon)) == fixed_volume_icon
@test_nowarn ModelingToolkitDesigner.view(design)
@test_nowarn ModelingToolkitDesigner.view(design, false)
| ModelingToolkitDesigner | https://github.com/bradcarman/ModelingToolkitDesigner.jl.git |
|
[
"MIT"
] | 1.3.0 | 91d51d3b38c103a0e0820f2e4419812efdd7e7e5 | code | 116 | connect(stp.output, src.input)
connect(src.port, res.port_a)
connect(vol.port, res.port_b)
connect(src.port, fluid)
| ModelingToolkitDesigner | https://github.com/bradcarman/ModelingToolkitDesigner.jl.git |
|
[
"MIT"
] | 1.3.0 | 91d51d3b38c103a0e0820f2e4419812efdd7e7e5 | docs | 8176 | # ModelingToolkitDesigner.jl
The ModelingToolkitDesigner.jl package is a helper tool for visualizing and editing ModelingToolkit.jl system connections.
# Updates
## v1.3
- updating to ModelingToolkit v9
## v1.1.0
- easier component selection (one only needs to click inside the boarder box)
## v1.0.0
- added icon rotation feature
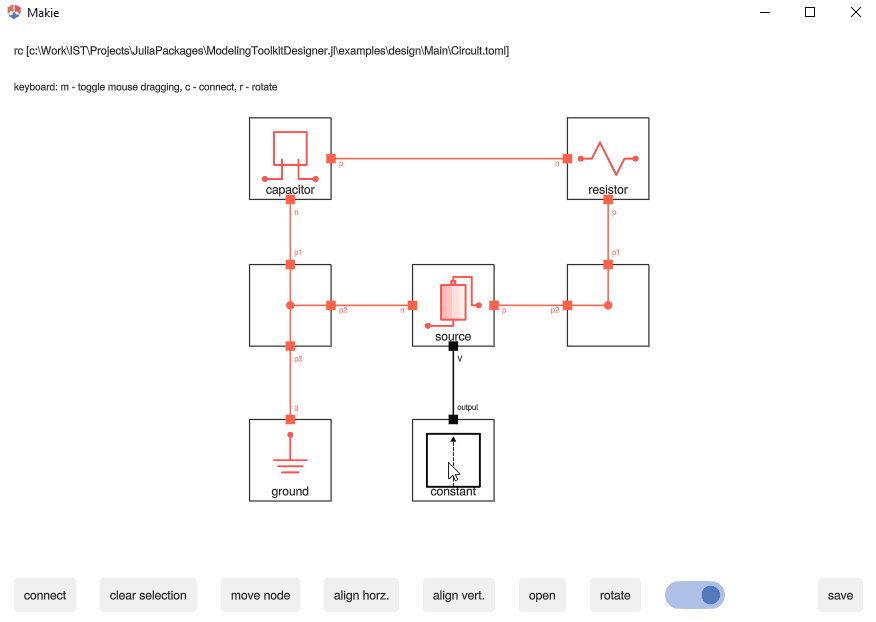
- added keyboard commands
- m: after selecting a component, use the `m` key to turn dragging on. This can be easier then clicking and dragging.
- c: connect
- r: rotate
## v0.3.0
- Connector nodes are now correctly positioned around the component with natural ordering
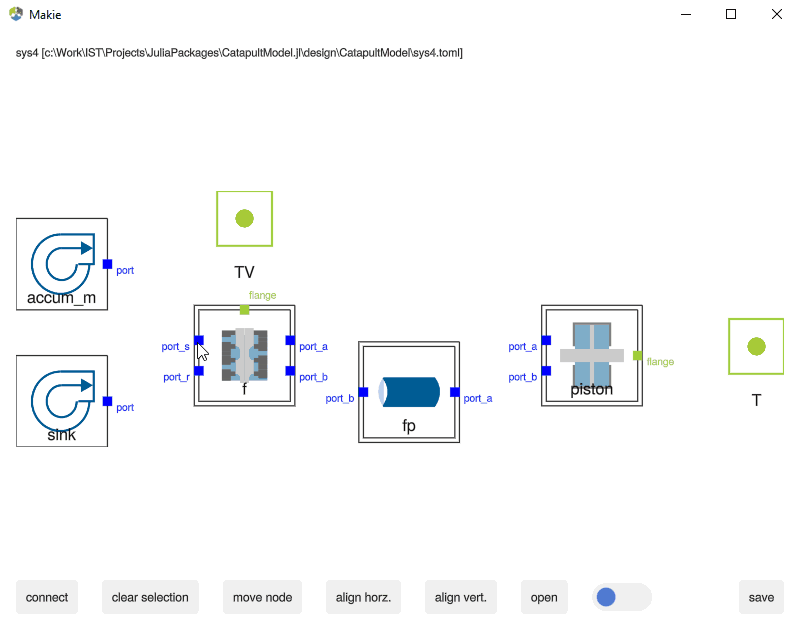
## v0.2.0
- Settings Files (*.toml) Updates/Breaking Changes
- icon rotation now saved with key `r`, previously was `wall` which was not the best name
- connectors nodes now saved with an underscore: `_name`, this helps prevent a collision with nodes named `x`, `y`, or `r`
## Examples
Examples can be found in the "examples" folder.
### Hydraulic Example

### Electrical Example

### Mechanical Example

# Tutorial
Let's start with a simple hydraulic system with no connections defined yet...
```julia
using ModelingToolkit
using ModelingToolkitDesigner
using GLMakie
import ModelingToolkitStandardLibrary.Hydraulic.IsothermalCompressible as IC
import ModelingToolkitStandardLibrary.Blocks as B
@parameters t
@component function system(; name)
pars = []
systems = @named begin
stp = B.Step(;height = 10e5, start_time = 0.005)
src = IC.InputSource(;p_int=0)
vol = IC.FixedVolume(;p_int=0, vol=10.0)
res = IC.Pipe(5; p_int=0, area=0.01, length=500.0)
end
eqs = Equation[]
ODESystem(eqs, t, [], pars; name, systems)
end
@named sys = system()
```
Then we can visualize the system using `ODESystemDesign()` and the `view()` functions
```julia
path = joinpath(@__DIR__, "design") # folder where visualization info is saved and retrieved
design = ODESystemDesign(sys, path);
ModelingToolkitDesigner.view(design)
```
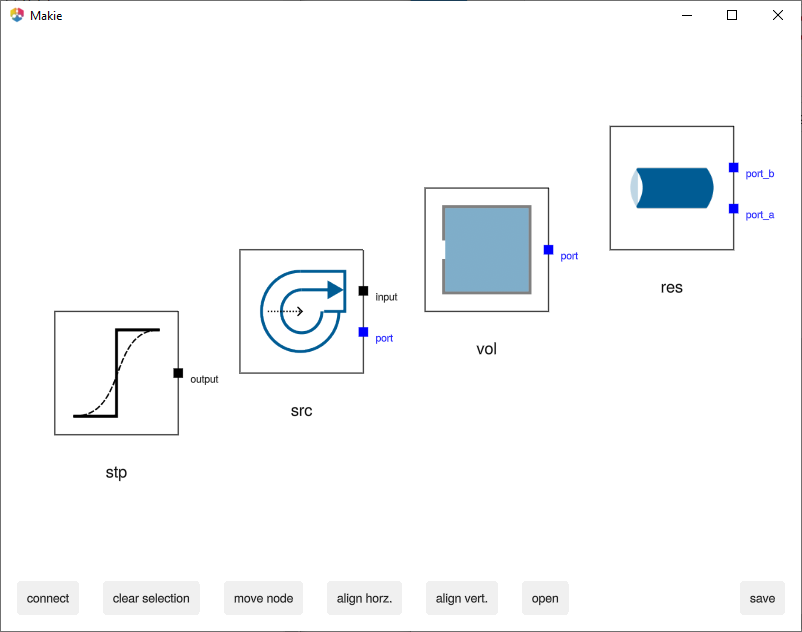
Components can then be positioned in several ways:
- keyboard: select component and then use up, down, left, right keys
- mouse: click and drag (or click and then `m` key)
- alignment: select and align horrizontal or vertial with respective buttons
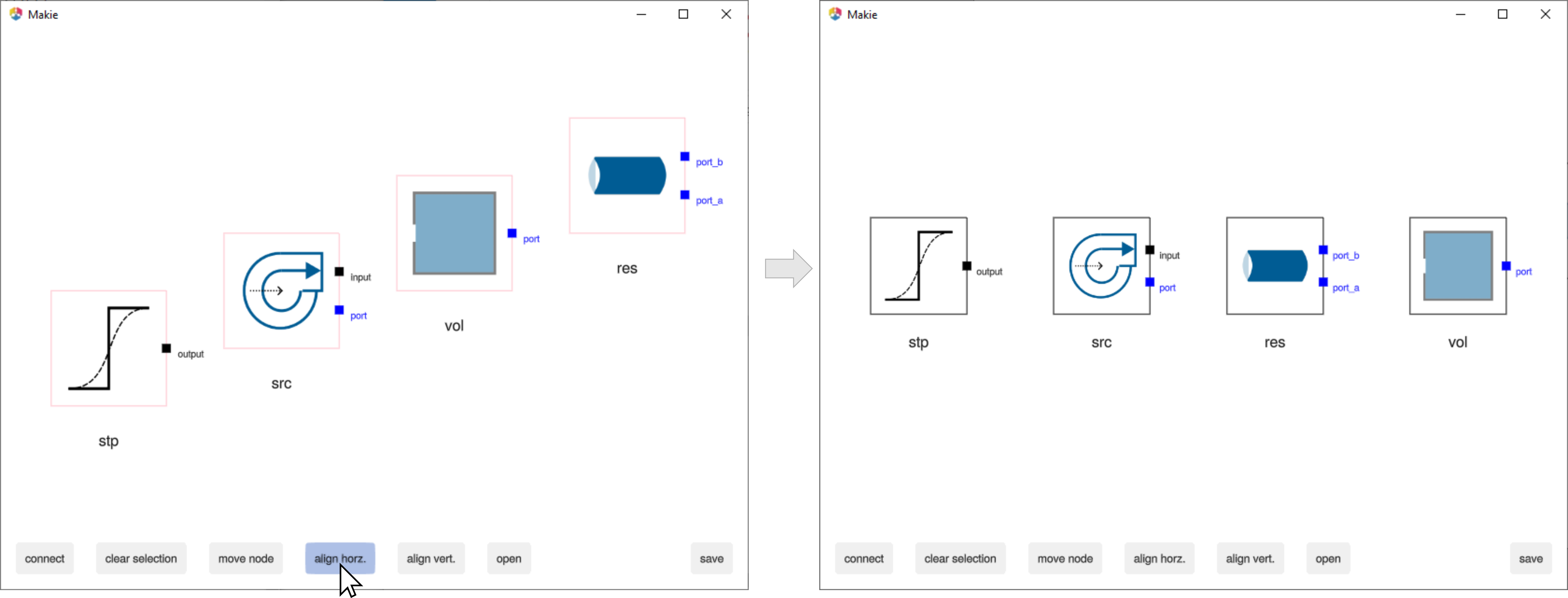
Nodes/Connectors can be positioned by selecting with the mouse and using the __move node__ button. *Note: Components and Nodes can be de-selected by right clicking anywhere or using the button* __clear selection__
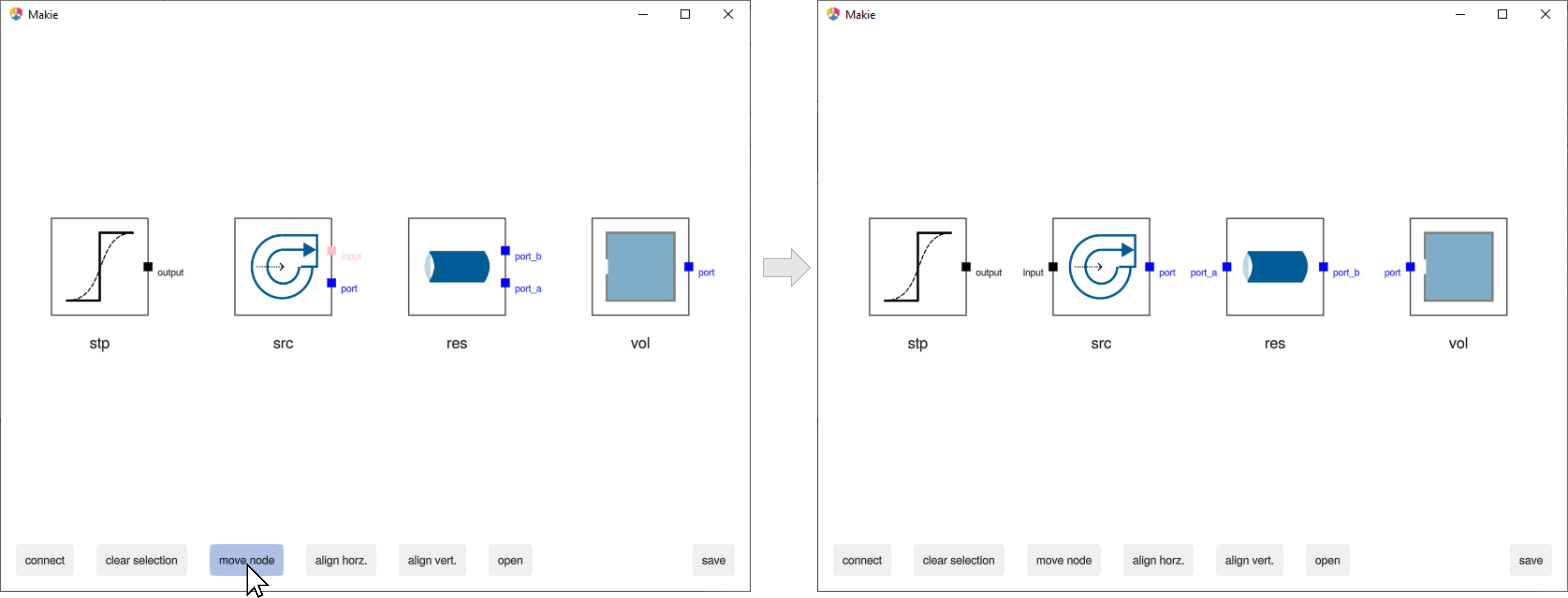
Connections can then be made by clicking 2 nodes and using the __connect__ button or the `c` key. One can then click the __save__ button which will store the visualization information in the `path` location in a `.toml` format as well as the connection code in `.jl` format.
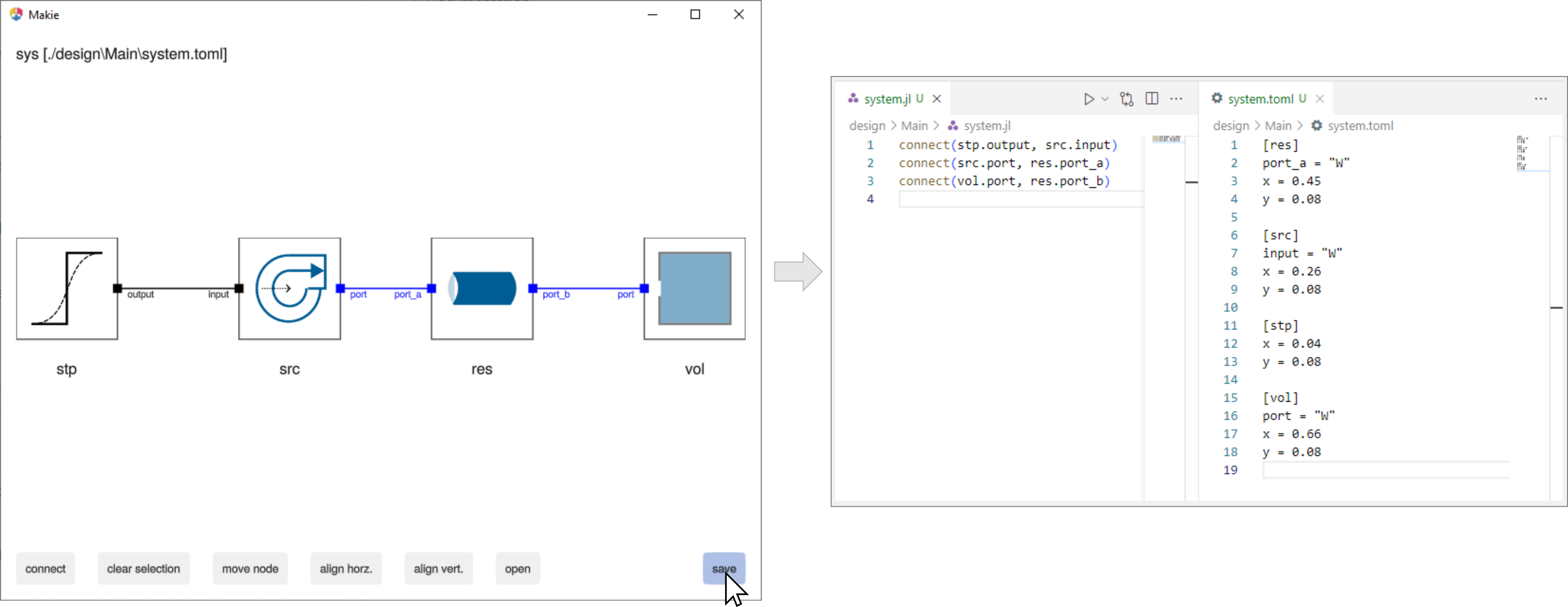
The connection code can also be obtained with the `connection_code()` function
```julia
julia> connection_code(design)
connect(stp.output, src.input)
connect(src.port, res.port_a)
connect(vol.port, res.port_b)
```
After the original `system()` component function is updated with the connection equations, the connections will be re-drawn automatically when using `ModelingToolkitDesigner.view()`. *Note: CairoMakie vector based images can also be generated by passing `false` to the 2nd argument of `view()` (i.e. the `interactive` variable).*
# Hierarchy
If a component has a double lined box then it is possible to "look under the hood". Simply select the component and click __open__.
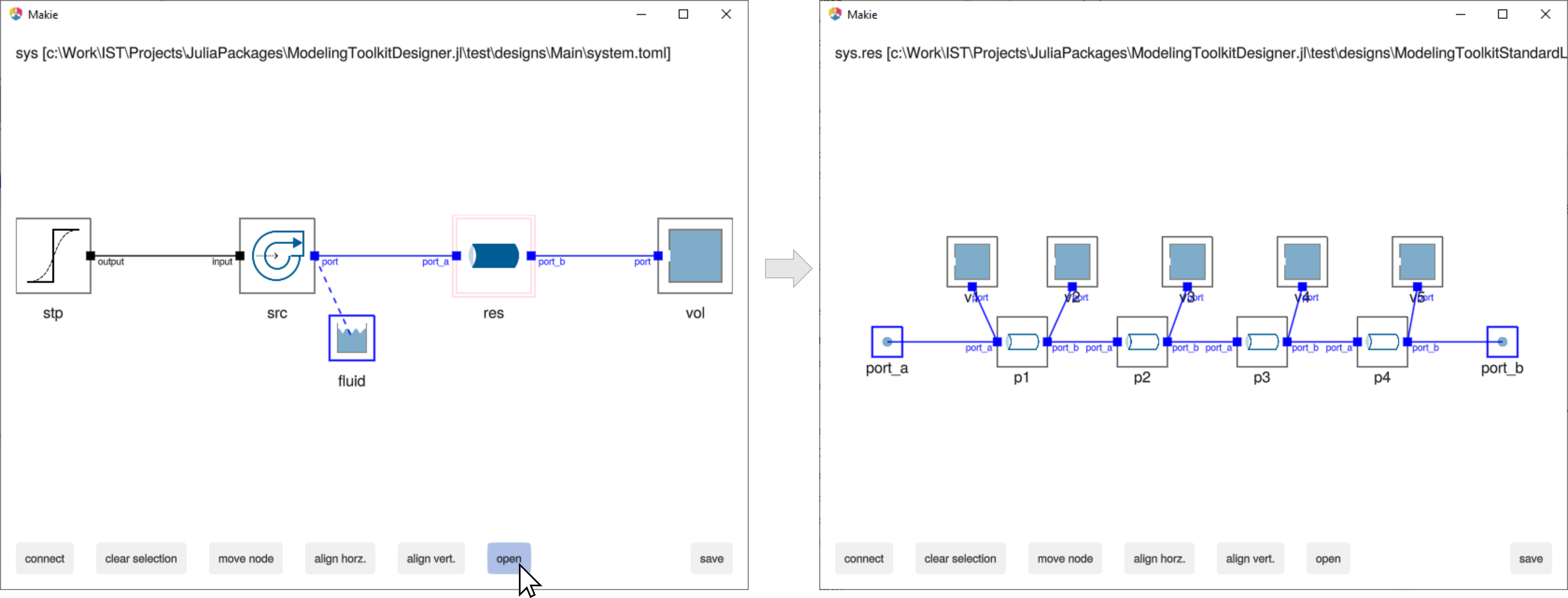
Edits made to sub-components can be saved and loaded directly or indirectly.
# Pass Thrus
To generate more esthetic diagrams, one can use as special kind of component called a `PassThru`, which simply defines 2 or more connected `connectors` to serve as a corner, tee, or any other junction. Simply define a component function starting with `PassThru` and ModelingToolkitDesigner.jl will recognize it as a special component type. For example for a corner with 2 connection points:
```julia
@component function PassThru2(;name)
@variables t
systems = @named begin
p1 = Pin()
p2 = Pin()
end
eqs = [
connect(p1, p2)
]
return ODESystem(eqs, t, [], []; name, systems)
end
```
And for a tee with 3 connection points
```julia
@component function PassThru3(;name)
@variables t
systems = @named begin
p1 = Pin()
p2 = Pin()
p3 = Pin()
end
eqs = [
connect(p1, p2, p3)
]
return ODESystem(eqs, t, [], []; name, systems)
end
```
Adding these components to your system will then allow for corners, tees, etc. to be created. When editing is complete, use the toggle switch to hide the `PassThru` details, showing a more esthetic connection diagram.
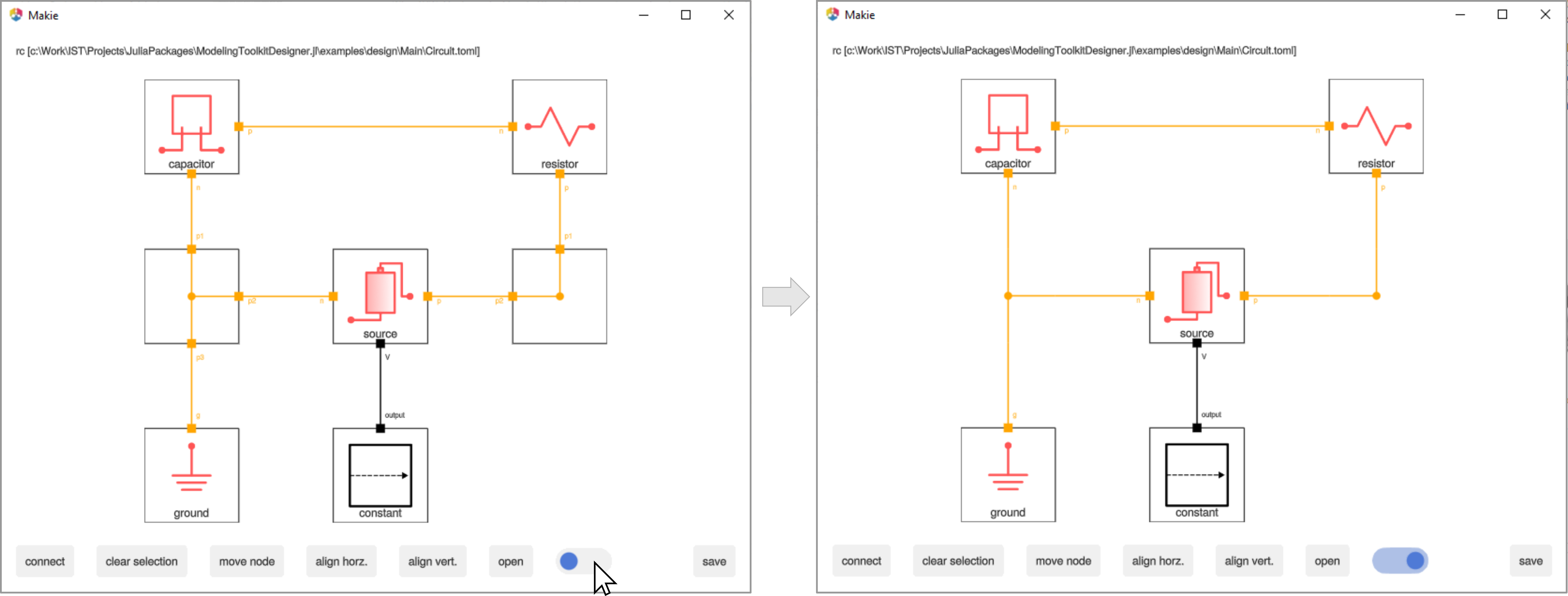
# Icons
ModelingToolkitDesigner.jl comes with icons for the ModelingToolkitStandardLibrary.jl pre-loaded. For custom components, icons are loaded from either the `path` variable supplied to `ODESystemDesign()` or from an `icons` folder of the package namespace. To find the paths ModelingToolkitDesign.jl is searching, run the following on the component of interest (for example `sys.vol`)
```julia
julia> println.(ModelingToolkitDesigner.get_icons(sys.vol, path));
...\ModelingToolkitDesigner.jl\examples\design\ModelingToolkitStandardLibrary\Hydraulic\IsothermalCompressible\FixedVolume.png
...\ModelingToolkitStandardLibrary.jl\icons\ModelingToolkitStandardLibrary\Hydraulic\IsothermalCompressible\FixedVolume.png
...\ModelingToolkitDesigner.jl\icons\ModelingToolkitStandardLibrary\Hydraulic\IsothermalCompressible\FixedVolume.png
```
The first file location comes from the `path` variable. The second is looking for a folder `icons` in the component parent package, in this case `ModelingToolkitStandardLibrary`, and the third path is from the `icons` folder of this `ModelingToolkitDesigner` package. The first real file is the chosen icon load path. Feel free to contribute icons here for any other public component libraries.
## Icon Rotation
Rotate an icon using the button or key `r`. The icon rotation is controlled by the `r` atribute in the saved `.toml` design file. The default direction is "E" for east. The image direciton can change to any "N","S","E","W". For example, to rotate the capacitor icon by -90 degrees (i.e. from "E" to "N") simply edit the design file as
```
[capacitor]
_n = "S"
x = 0.51
y = 0.29
r = "N"
```
# Colors
ModelingToolkitDesigner.jl colors the connections based on `ModelingToolkitDesigner.design_colors`. Colors for the ModelingToolkitStandardLibrary.jl are already loaded. To add a custom connector color, simply use `add_color(system::ODESystem, color::Symbol)` where `system` is a reference to the connector (e.g. `sys.vol.port`) and `color` is a named color from [Colors.jl](https://juliagraphics.github.io/Colors.jl/stable/namedcolors/).
# TODO
- Finish adding icons for the ModelingToolkitStandardLibrary.jl
- Improve text positioning and fontsize
- How to include connection equations automatically, maybe implement the `input` macro
- Provide `PassThru`'s without requiring user to add `PassThru` components to ODESystem
- Add documentation | ModelingToolkitDesigner | https://github.com/bradcarman/ModelingToolkitDesigner.jl.git |
|
[
"MIT",
"BSL-1.0"
] | 0.5.0 | e96812c38a7ca1c013d35c9515f05a1d023e2191 | code | 852 | using SoapySDR
using Documenter
DocMeta.setdocmeta!(SoapySDR, :DocTestSetup, :(using SoapySDR); recursive = true)
makedocs(;
modules = [SoapySDR],
authors = "JuliaTelecom and contributors",
repo = "https://github.com/JuliaTelecom/SoapySDR.jl/blob/{commit}{path}#{line}",
sitename = "SoapySDR.jl",
format = Documenter.HTML(;
prettyurls = get(ENV, "CI", "false") == "true",
canonical = "https://JuliaTelecom.github.io/SoapySDR.jl",
assets = String[],
),
pages = [
"Home" => "index.md",
"Tutorial" => "tutorial.md",
"Loading Drivers" => "drivers.md",
"High Level API" => "highlevel.md",
"Low Level API" => "lowlevel.md",
"Troubleshooting" => "troubleshooting.md",
],
)
deploydocs(; repo = "github.com/JuliaTelecom/SoapySDR.jl", devbranch = "main")
| SoapySDR | https://github.com/JuliaTelecom/SoapySDR.jl.git |
|
[
"MIT",
"BSL-1.0"
] | 0.5.0 | e96812c38a7ca1c013d35c9515f05a1d023e2191 | code | 2029 | # This script shows how to use the direct buffer access API with the RTLSDR
# and validate acess using its internal testmode
using SoapySDR
using SoapyRTLSDR_jll
SoapySDR.versioninfo()
function dma_test()
# open the first device
devs = Devices()
dev_args = devs[1]
dev = Device(dev_args)
# get the RX channel
chan = dev.rx[1]
# enable the test pattern so we can validate the type conversions
SoapySDR.SoapySDRDevice_writeSetting(dev, "testmode", "true")
native_format = chan.native_stream_format
# open RX stream
stream = SDRStream(native_format, [chan])
for rate in SoapySDR.list_sample_rates(chan)
println("Testing sample rate:", rate)
chan.sample_rate = rate
SoapySDR.activate!(stream)
# acquire buffers using the low-level API
buffs = Ptr{native_format}[C_NULL]
bytes = 0
total_bytes = 0
timeout_count = 0
overflow_count = 0
buf_ct = stream.num_direct_access_buffers
println("Receiving data")
time = @elapsed for i = 1:buf_ct*3 # cycle through all buffers three times
err, handle, flags, timeNs =
SoapySDR.SoapySDRDevice_acquireReadBuffer(dev, stream, buffs, 1000000)
if err == SOAPY_SDR_TIMEOUT
timeout_count += 1
elseif err == SOAPY_SDR_OVERFLOW
overflow_count += 1
end
arr = unsafe_wrap(Array{native_format}, buffs[1], stream.mtu)
# check the count
for j in eachindex(arr)
@assert imag(arr[j]) - real(arr[j]) == 1
end
SoapySDR.SoapySDRDevice_releaseReadBuffer(dev, stream, handle)
total_bytes += stream.mtu * sizeof(native_format)
end
println("Data rate: $(Base.format_bytes(total_bytes / time))/s")
println("Timeout count:", timeout_count)
println("Overflow count:", overflow_count)
SoapySDR.deactivate!(stream)
end
end
dma_test()
| SoapySDR | https://github.com/JuliaTelecom/SoapySDR.jl.git |
|
[
"MIT",
"BSL-1.0"
] | 0.5.0 | e96812c38a7ca1c013d35c9515f05a1d023e2191 | code | 2639 | using FFTW
using PyPlot
using DSP
using WAV
function downsample(data, M)
order = 35 # filter order
d = 0.01 # transition band
coeffs = remez(order, [0, 1 / (M * 2) - d, 1 / (M * 2) + d, 0.5], [1, 0])
return (decimate(filt(coeffs, [1], data), M), coeffs, [1])
end
function decimate(data, M)
return data[1:M:end]
end
function discriminator(data, M)
# Limiter
data = data ./ abs.(data)
# differentiator
(data2, b2, a2) = differentiator(data, M)
# complex conj delay
(ds, b, a) = delay((conj.(data2)), (M - 1) / 2.0, 80)
data_mod = ds .* data2
return (imag.(data_mod), b, a, b2, a2)
end
function differentiator(data, M)
b = remez(M, [0, 0.5], [1.0], filter_type = RemezFilterType(2))
return (filt(b, [1], data), b, [1])
end
function delay(data, D, o)
a = 0.1
b = [sin.(a * (n - D)) / (a * (n - D)) + 0im for n = 0:o-1]
c = filt(b, a, data)
return (c, b, [1])
end
function deemphasis(data, t, f)
T = 1 / f
al = 1 / tan(T / (2 * t))
b = [1, 1]
a = [1 + al, 1 - al]
return (filt(b, a, data), b, a)
end
function lowPassFilter(data, f, pb, sb)
fp = pb / f
ft = sb / f
order = 35
coeffs = remez(order, [0, fp, ft, 0.5], [1, 0])
return (filt(coeffs, [1], data), coeffs, [1])
end
function plotTimeFreq(storeFft, fs, f0)
w, h = figaspect(0.33)
figure(figsize = [w, h])
minF = (f0 - fs / 2) ./ 1e6
maxF = (f0 + fs / 2) ./ 1e6
maxT = 1 / fs * size(storeFft)[1] * size(storeFft)[2]
imshow(storeFft, extent = [minF, maxF, 0, maxT], aspect = "auto")
xlabel("Frequnecy (MHz)")
ylabel("Time (s)")
cb = colorbar()
cb[:set_label]("Magnitude (dB)")
end
function plotTime(data, fs)
w, h = figaspect(0.33)
figure(figsize = [w, h])
maxT = 1 / fs * size(data)[1]
t = range(0, maxT, length = size(data)[1])
plot(t, data, linewidth = 0.1)
xlim([0, maxT])
xlabel("Time (s)")
ylabel("Amplitude")
end
function plotIq(data)
figure()
scatter(real.(data), imag.(data), s = 10)
xlabel("In-phase")
ylabel("Quadrature")
title("IQ data")
end
function fmDemod(data, fs)
# downsample
fs2 = 256e3
M = Int(fs / fs2)
(data3, b3, a3) = downsample(data, M)
(data4, b4, a4, b42, a42) = discriminator(data3, 10)
t = 75e-6
(data5, b5, a5) = deemphasis(data4, t, fs2)
(data6, b6, a6) = lowPassFilter(data5, fs2, 15e3, 18e3)
fs3 = 64e3
M = Int(fs2 / fs3)
(data7, b7, a7) = downsample(data6, M)
maxval = abs(data7[argmax(abs.(data7))])
data8 = data7 ./ maxval
return (data8, fs3)
return (data7, fs3)
end
| SoapySDR | https://github.com/JuliaTelecom/SoapySDR.jl.git |
|
[
"MIT",
"BSL-1.0"
] | 0.5.0 | e96812c38a7ca1c013d35c9515f05a1d023e2191 | code | 4805 | using Printf
using FFTW
using PyPlot
using DSP
using SoapySDR
# Don't forget to add/import a device-specific plugin package!
# using xtrx_jll
# using SoapyLMS7_jll
# using SoapyRTLSDR_jll
# using SoapyPlutoSDR_jll
# using SoapyUHD_jll
include("fmDemod.jl")
# enumerate devices
(kwargs, sz) = SoapySDR.SoapySDRDevice_enumerate()
t = unsafe_load(kwargs)
for i = 1:Int(sz[])
@printf "\nnumber of results in device = %i\n" t.size
@printf "Found device #%d: " i
keys = unsafe_string.(unsafe_wrap(Array, t.keys, t.size))
vals = unsafe_string.(unsafe_wrap(Array, t.vals, t.size))
for j = 1:t.size
@printf "%s=%s, " keys[j] vals[j]
end
@printf "\n"
end
@printf "\nnumber of devices = %i\n" Int(sz[])
SoapySDR.SoapySDRKwargs_clear(kwargs)
# create device instance
# args can be user defined or from the enumeration result
sdr = SoapySDR.SoapySDRDevice_make(kwargs)
if (unsafe_load(sdr) == C_NULL)
@printf "SoapySDRDevice_make fail: %s\n" unsafe_string(
SoapySDR.SoapySDRDevice_lastError(),
)
end
# query device info
(name, sz) = SoapySDR.SoapySDRDevice_listAntennas(sdr, SoapySDR.SOAPY_SDR_RX, 0)
@printf "Rx antennas: "
for i = 1:Int(sz[])
@printf "%s, " unsafe_string.(unsafe_wrap(Array, name, Int(sz[])))[i]
end
@printf "\n"
(name2, sz2) = SoapySDR.SoapySDRDevice_listGains(sdr, SoapySDR.SOAPY_SDR_RX, 0)
@printf "Rx gains: "
for i = 1:Int(sz[])
@printf "%s, " unsafe_string.(unsafe_wrap(Array, name2, Int(sz2[])))[i]
end
@printf "\n"
(ranges, sz) = SoapySDR.SoapySDRDevice_getFrequencyRange(sdr, SoapySDR.SOAPY_SDR_RX, 0)
@printf "Rx freq ranges: "
for i = 1:Int(sz[])
range = unsafe_wrap(Array, ranges, Int(sz[]))[i]
@printf "[%g Hz -> %g Hz], " range.minimum range.maximum
end
@printf "\n"
# apply settings
#sampRate = 1024e3
sampRate = 2048e3
#sampRate = 512e3
if (SoapySDR.SoapySDRDevice_setSampleRate(sdr, SoapySDR.SOAPY_SDR_RX, 0, sampRate) != 0)
@printf "setSampleRate fail: %s\n" unsafe_string(SoapySDR.SoapySDRDevice_lastError())
end
#f0 = 103.3e6
f0 = 104.1e6
#f0 = 938.2e6
if (SoapySDR.SoapySDRDevice_setFrequency(sdr, SoapySDR.SOAPY_SDR_RX, 0, f0, C_NULL) != 0)
@printf "setFrequency fail: %s\n" unsafe_string(SoapySDR.SoapySDRDevice_lastError())
end
# set up a stream (complex floats)
rxStream = SoapySDR.SoapySDRStream()
if (
SoapySDR.SoapySDRDevice_setupStream(
sdr,
SoapySDR.SOAPY_SDR_RX,
SoapySDR.SOAPY_SDR_CF32,
C_NULL,
0,
SoapySDR.KWArgs(),
) != 0
)
@printf "setupStream fail: %s\n" unsafe_string(SoapySDR.SoapySDRDevice_lastError())
end
# start streaming
SoapySDR.SoapySDRDevice_activateStream(sdr, pointer_from_objref(rxStream), 0, 0, 0)
# create a re-usable buffer for rx samples
buffsz = 1024
buff = Array{ComplexF32}(undef, buffsz)
# receive some samples
timeS = 15
timeSamp = Int(floor(timeS * sampRate / buffsz))
storeIq = zeros(ComplexF32, buffsz * timeSamp)
flags = Ref{Cint}()
timeNs = Ref{Clonglong}()
buffs = [buff]
#storeFft = zeros(timeSamp, buffsz)
storeBuff = zeros(ComplexF32, timeSamp, buffsz)
for i = 1:timeSamp
nelem, flags, timeNs = SoapySDR.SoapySDRDevice_readStream(
sdr,
pointer_from_objref(rxStream),
Ref(pointer(buff)),
buffsz,
100000,
)
local storeBuff[i, :] = buff
end
b = blackman(20)
storeFft = zeros(timeSamp, buffsz)
for i = 1:size(storeBuff)[1]
local storeFft[i, :] = 20 .* log10.(abs.(fftshift(fft(storeBuff[i, :]))))
end
# get IQ array
storeIq = Array(reshape(storeBuff', :, size(storeBuff)[1] * size(storeBuff)[2])')[:]
# shutdown the stream
SoapySDR.SoapySDRDevice_deactivateStream(sdr, pointer_from_objref(rxStream), 0, 0) # stop streaming
SoapySDR.SoapySDRDevice_closeStream(sdr, pointer_from_objref(rxStream))
SoapySDR.SoapySDRDevice_unmake(sdr)
function plotTimeFreq(storeFft, fs, f0)
w, h = figaspect(0.5)
figure(figsize = [w, h])
minF = (f0 - fs / 2) ./ 1e6
maxF = (f0 + fs / 2) ./ 1e6
maxT = 1 / fs * size(storeFft)[1] * size(storeFft)[2]
imshow(storeFft, extent = [minF, maxF, 0, maxT], aspect = "auto")
xlabel("Frequnecy (MHz)")
ylabel("Time (s)")
cb = colorbar()
cb[:set_label]("Magnitude (dB)")
end
function plotTime(data, fs)
w, h = figaspect(0.25)
figure(figsize = [w, h])
maxT = 1 / fs * size(data)[1]
t = range(0, maxT, length = size(data)[1])
plot(t, data, linewidth = 0.1)
xlim([0, maxT])
xlabel("Time (s)")
ylabel("Amplitude")
end
function plotIq(data)
figure()
scatter(real.(data), imag.(data), s = 10)
xlabel("In-phase")
ylabel("Quadrature")
title("IQ data")
end
plotIq(storeIq[1:10000])
plotTimeFreq(storeFft, sampRate, f0)
(data, fs) = fmDemod(storeIq, sampRate)
plotTime(data, fs)
wavwrite(data, "demod.wav", Fs = fs)
| SoapySDR | https://github.com/JuliaTelecom/SoapySDR.jl.git |
|
[
"MIT",
"BSL-1.0"
] | 0.5.0 | e96812c38a7ca1c013d35c9515f05a1d023e2191 | code | 2315 | using Printf
using FFTW
using PyPlot
using DSP
using SoapySDR
using Unitful
# Don't forget to add/import a device-specific plugin package!
# using xtrx_jll
# using SoapyLMS7_jll
# using SoapyRTLSDR_jll
# using SoapyPlutoSDR_jll
# using SoapyUHD_jll
include("fmDemod.jl")
include("highlevel_dump_devices.jl")
devs = Devices()
sdr = Device(devs[1])
rx1 = sdr.rx[1]
# setup AGC if available
#rx1.gain_mode = true
sampRate = 2.048e6
rx1.sample_rate = sampRate * u"Hz"
f0 = 104.1e6
rx1.frequency = f0 * u"Hz"
# set up a stream (complex floats)
rxStream = SoapySDR.Stream(ComplexF32, [rx1])
# start streaming
# create a re-usable buffer for rx samples
buffsz = 1024
buff = Array{ComplexF32}(undef, buffsz)
# receive some samples
timeS = 15
timeSamp = Int(floor(timeS * sampRate / buffsz))
storeIq = zeros(ComplexF32, buffsz * timeSamp)
#storeFft = zeros(timeSamp, buffsz)
storeBuff = zeros(ComplexF32, timeSamp, buffsz)
# Enable ther stream
SoapySDR.activate!(rxStream)
for i = 1:timeSamp
read!(rxStream, [buff])
storeBuff[i, :] = buff
end
b = blackman(20)
storeFft = zeros(timeSamp, buffsz)
for i = 1:size(storeBuff)[1]
local storeFft[i, :] = 20 .* log10.(abs.(fftshift(fft(storeBuff[i, :]))))
end
# get IQ array
storeIq = Array(reshape(storeBuff', :, size(storeBuff)[1] * size(storeBuff)[2])')[:]
function plotTimeFreq(storeFft, fs, f0)
w, h = figaspect(0.5)
figure(figsize = [w, h])
minF = (f0 - fs / 2) ./ 1e6
maxF = (f0 + fs / 2) ./ 1e6
maxT = 1 / fs * size(storeFft)[1] * size(storeFft)[2]
imshow(storeFft, extent = [minF, maxF, 0, maxT], aspect = "auto")
xlabel("Frequency (MHz)")
ylabel("Time (s)")
cb = colorbar()
cb[:set_label]("Magnitude (dB)")
end
function plotTime(data, fs)
w, h = figaspect(0.25)
figure(figsize = [w, h])
maxT = 1 / fs * size(data)[1]
t = range(0, maxT, length = size(data)[1])
plot(t, data, linewidth = 0.1)
xlim([0, maxT])
xlabel("Time (s)")
ylabel("Amplitude")
end
function plotIq(data)
figure()
scatter(real.(data), imag.(data), s = 10)
xlabel("In-phase")
ylabel("Quadrature")
title("IQ data")
end
plotIq(storeIq[1:10000])
plotTimeFreq(storeFft, sampRate, f0)
(data, fs) = fmDemod(storeIq, sampRate)
plotTime(data, fs)
wavwrite(data, "demod.wav", Fs = fs)
| SoapySDR | https://github.com/JuliaTelecom/SoapySDR.jl.git |
|
[
"MIT",
"BSL-1.0"
] | 0.5.0 | e96812c38a7ca1c013d35c9515f05a1d023e2191 | code | 468 | using SoapySDR
# Don't forget to add/import a device-specific plugin package!
# using xtrx_jll
# using SoapyLMS7_jll
# using SoapyRTLSDR_jll
for (didx, dev) in enumerate(Devices())
@info("device", dev, idx = didx)
dev = Device(dev)
@info("TX channels:")
for (idx, tx_channel) in enumerate(dev.tx)
display(tx_channel)
end
@info("RX channels:")
for (idx, rx_channel) in enumerate(dev.rx)
display(rx_channel)
end
end
| SoapySDR | https://github.com/JuliaTelecom/SoapySDR.jl.git |
|
[
"MIT",
"BSL-1.0"
] | 0.5.0 | e96812c38a7ca1c013d35c9515f05a1d023e2191 | code | 2572 | using SoapySDR
# Don't forget to add/import a device-specific plugin package!
# using xtrx_jll
# using SoapyLMS7_jll
# using SoapyRTLSDR_jll
# using SoapyPlutoSDR_jll
# using SoapyUHD_jll
# Or we can load OS provided modules or custom modules
# with the SOAPY_SDR_PLUGIN_PATH environment variable:
# ENV["SOAPY_SDR_PLUGIN_PATH"]="/usr/lib/x86_64-linux-gnu/SoapySDR/modules0.8/"
# Get the Devices
devs = Devices()
# we can add arguments to the device constructor or filter multiple devices:
# devs[1]["refclk"] = "26000000"
# devs = filter(x -> haskey(x, "driver") && x["driver"] == "plutosdr", devs)
# Now open the first device and get the first RX and TX channel streams
dev = Device(devs[1])
c_tx = dev.tx[1]
c_rx = dev.rx[1]
# Configure channel with appropriate parameters. We choose to sneak into
# the very high end of the 2.4 GHz ISM band, above Wifi channel 14 (which
# itself is well outside of the typical US equipment range, which only goes
# up to channel 11, e.g. 2.473 GHz).
c_tx.bandwidth = 2u"MHz"
c_rx.bandwidth = 200u"kHz"
c_tx.frequency = 2.498u"GHz"
c_rx.frequency = 2.498u"GHz"
c_tx.gain = 52u"dB"
c_rx.gain = -2u"dB"
c_tx.sample_rate = 1u"MHz"
c_rx.sample_rate = 1u"MHz"
# Write out a sinusoid oscillating at 100KHz, lasting 10ms
t = (1:round(Int, 0.01 * 1e6)) ./ 1e6
data_tx = ComplexF32.(sin.(2π .* t .* 100e3), 0.0f0)
data_tx_zeros = zeros(ComplexF32, length(data_tx))
# Open both RX and TX streams
s_tx = SoapySDR.Stream(ComplexF32, [c_tx])
s_rx = SoapySDR.Stream(ComplexF32, [c_rx])
# Then, do the actual writing and reading!
function loopback_test(s_tx, s_rx, num_buffers)
# allocate some recieve buffers
data_rx_buffs = Vector{ComplexF32}[]
for _ = 1:num_buffers
push!(data_rx_buffs, Vector(undef, length(data_tx)))
end
SoapySDR.activate!.((s_tx, s_rx))
# Read/write `num_buffers`, writing zeros out except for one buffer.
for idx = 1:num_buffers
if idx == ceil(num_buffers / 2)
Base.write(s_tx, [data_tx])
else
Base.write(s_tx, [data_tx_zeros])
end
Base.read!(s_rx, [data_rx_buffs[idx]])
end
SoapySDR.deactivate!.((s_tx, s_rx))
return data_rx_buffs
end
# This should take about 100ms, since we're rx/tx'ing 10x buffers which should each be 10ms long.
loopback_test(s_tx, s_rx, 2)
data_rx_buffs = loopback_test(s_tx, s_rx, 3)
# Join all buffers together
data_rx = vcat(data_rx_buffs...)
# Should see a nice big spike of a sinusoid being transmitted in the middle
using Plots;
plotly(size = (750, 750));
plot(real.(data_rx));
| SoapySDR | https://github.com/JuliaTelecom/SoapySDR.jl.git |
|
[
"MIT",
"BSL-1.0"
] | 0.5.0 | e96812c38a7ca1c013d35c9515f05a1d023e2191 | code | 3359 | using Printf
using FFTW
using GLMakie
using DSP
using SoapySDR
using Unitful
using TimerOutputs
using Observables
# Don't forget to add/import a device-specific plugin package!
# using xtrx_jll
# using SoapyLMS7_jll
using SoapyRTLSDR_jll
# using SoapyPlutoSDR_jll
# using SoapyUHD_jll
include("highlevel_dump_devices.jl")
get_time_ms() = trunc(Int, time() * 1000)
function makie_fft(direct_buffer_access = true, timer_display = false)
devs = Devices()
sdr = Device(devs[1])
rx1 = sdr.rx[1]
sampRate = 2.048e6
rx1.sample_rate = sampRate * u"Hz"
# Enable automatic Gain Control
rx1.gain_mode = true
to = TimerOutput()
f0 = 104.1e6
rx1.frequency = f0 * u"Hz"
# set up a stream (complex floats)
format = rx1.native_stream_format
rxStream = SoapySDR.Stream(format, [rx1])
# create a re-usable buffer for rx samples
buffsz = rxStream.mtu
buff = Array{format}(undef, buffsz)
buffs = Ptr{format}[C_NULL] # pointer for direct buffer API
# receive some samples
timeS = 10
timeSamp = Int(floor(timeS * sampRate / buffsz))
decimator_factor = 16
storeFft = Observable(zeros(timeSamp, div(buffsz, decimator_factor)))
@info "initializing plot..."
fig = heatmap(storeFft)
display(fig)
@info "planning fft..."
fft_plan_a = plan_fft(buff)
last_plot = get_time_ms()
last_timeoutput = get_time_ms()
# If there is timing slack, we can sleep a bit to run event handlers
have_slack = true
# Enable ther stream
@info "streaming..."
SoapySDR.activate!(rxStream)
while true
@timeit to "Reading stream" begin
if !direct_buffer_access
read!(rxStream, (buff,))
else
err, handle, flags, timeNs =
SoapySDR.SoapySDRDevice_acquireReadBuffer(sdr, rxStream, buffs, 0)
if err == SoapySDR.SOAPY_SDR_TIMEOUT
sleep(0.001)
have_slack = true
continue # we don't have any data available yet, so loop
elseif err == SoapySDR.SOAPY_SDR_OVERFLOW
have_slack = false
err = buffsz # nothing to do, should be the MTU
end
@assert err > 0
buff = unsafe_wrap(Array{format}, buffs[1], (buffsz,))
SoapySDR.SoapySDRDevice_releaseReadBuffer(sdr, rxStream, handle)
end
end
@timeit to "Copying FFT data" storeFft[][2:end, :] .= storeFft[][1:end-1, :]
@timeit to "FFT" storeFft[][1, :] =
20 .* log10.(abs.(fftshift(fft_plan_a * buff)))[1:decimator_factor:end]
@timeit to "Plotting" begin
if have_slack && get_time_ms() - last_plot > 100 # 10 fps
storeFft[] = storeFft[]
last_plot = get_time_ms()
end
end
if have_slack && timer_display
@timeit to "Timer Display" begin
if get_time_ms() - last_timeoutput > 3000
show(to)
last_timeoutput = get_time_ms()
end
end
end
@timeit to "GC" begin
have_slack && GC.gc(false)
end
have_slack && sleep(0.01) # give some time for Makie event handlers
end
end
makie_fft()
| SoapySDR | https://github.com/JuliaTelecom/SoapySDR.jl.git |
|
[
"MIT",
"BSL-1.0"
] | 0.5.0 | e96812c38a7ca1c013d35c9515f05a1d023e2191 | code | 925 | using SoapySDR, SoapyRTLSDR_jll
# Here we want to test the behavior in a loop, to ensure
# that we can block on buffer overflow conditions, and
# handle partial reads, measure latnecy, etc
function rapid_read()
dev = open(Devices()[1])
rx_chan = dev.rx
rx_stream = SoapySDR.Stream(rx_chan)
@show rx_stream.mtu
SoapySDR.activate!(rx_stream)
bufs = [Vector{SoapySDR.streamtype(rx_stream)}(undef, 1_000_000) for i = 1:2]
flip = true
while true
# double buffer
flip = !flip
current_buff = bufs[Int(flip)+1]
prev_buff = bufs[Int(!flip)+1]
read!(rx_stream, [current_buff])
# sanity checks?
#nequal = 0
#for i in eachindex(current_buff.bufs)
# nequal += Int(current_buff.bufs[1][i] == prev_buff.bufs[1][i])
#end
#@show current_buff.timens
#@show nequal, current_buff.timens, delta_t
end
end
| SoapySDR | https://github.com/JuliaTelecom/SoapySDR.jl.git |
|
[
"MIT",
"BSL-1.0"
] | 0.5.0 | e96812c38a7ca1c013d35c9515f05a1d023e2191 | code | 1062 | #! /usr/bin/env julia
using soapysdr_jll
using Clang.Generators
include_dir = joinpath(soapysdr_jll.artifact_dir, "include") |> normpath
clang_dir = joinpath(include_dir, "clang-c")
options = load_options(joinpath(@__DIR__, "generator.toml"))
@show options
# add compiler flags, e.g. "-DXXXXXXXXX"
args = get_default_args()
push!(args, "-I$include_dir")
@show args
headers = [
joinpath(include_dir, "SoapySDR", header) for
header in readdir(joinpath(include_dir, "SoapySDR")) if endswith(header, ".h")
]
@show headers
@show basename.(headers)
# there is also an experimental `detect_headers` function for auto-detecting top-level headers in the directory
# headers = detect_headers(clang_dir, args)
filter!(s -> basename(s) ∉ ["Config.h"], headers) # Deivce is hand-wrapped and COnfig is not needed
# A few macros that don't translate well, and not applicable
options["general"]["output_ignorelist"] = ["SOAPY_SDR_API", "SOAPY_SDR_LOCAL"]
# create context
@show options
ctx = create_context(headers, args, options)
# run generator
build!(ctx)
| SoapySDR | https://github.com/JuliaTelecom/SoapySDR.jl.git |
|
[
"MIT",
"BSL-1.0"
] | 0.5.0 | e96812c38a7ca1c013d35c9515f05a1d023e2191 | code | 1221 | """
`SoapySDR.Modules` provides a modules for loading SoapySDR modules via paths.
This is an alternative to environmental variables and dlopen.
"""
module Modules
using ..SoapySDR
function get_root_path()
ptr = SoapySDR.SoapySDR_getRootPath()
ptr == C_NULL ? "" : unsafe_string(ptr)
end
function list_search_paths()
len = Ref{Csize_t}()
ptr = SoapySDR.SoapySDR_listSearchPaths(len)
SoapySDR.StringList(ptr, len[])
end
function list()
len = Ref{Csize_t}()
ptr = SoapySDR.SoapySDR_listModules(len)
SoapySDR.StringList(ptr, len[])
end
function list_in_path(path)
len = Ref{Csize_t}()
ptr = SoapySDR.SoapySDR_listModulesPath(path, len)
SoapySDR.StringList(ptr, len[])
end
function load_module(path)
ptr = SoapySDR.SoapySDR_loadModule(path)
ptr == C_NULL ? "" : unsafe_string(ptr)
end
function get_module_version(path)
ptr = SoapySDR.SoapySDR_getModuleVersion(path)
ptr == C_NULL ? "" : unsafe_string(ptr)
end
function unload_module(path)
ptr = SoapySDR.SoapySDR_unloadModule(path)
ptr == C_NULL ? "" : unsafe_string(ptr)
end
function load()
SoapySDR.SoapySDR_loadModules()
end
function unload()
SoapySDR.SoapySDR_unloadModules()
end
end
| SoapySDR | https://github.com/JuliaTelecom/SoapySDR.jl.git |
|
[
"MIT",
"BSL-1.0"
] | 0.5.0 | e96812c38a7ca1c013d35c9515f05a1d023e2191 | code | 909 | module SoapySDR
using soapysdr_jll
const lib = soapysdr_jll.libsoapysdr
const soapysdr = soapysdr_jll.libsoapysdr
using CEnum
using Intervals
using Unitful
using Unitful.DefaultSymbols
const dB = u"dB"
const GC = Base.GC
export @u_str
include("error.jl")
include("lowlevel/auto_wrap.jl")
include("unithelpers.jl")
include("typemap.jl")
include("typewrappers.jl")
include("highlevel.jl")
include("functionwraps.jl")
include("logger.jl")
include("version.jl")
include("Modules.jl")
const SDRStream = Stream
export SDRStream,
SOAPY_SDR_TX,
SOAPY_SDR_RX,
SOAPY_SDR_END_BURST,
SOAPY_SDR_HAS_TIME,
SOAPY_SDR_END_ABRUPT,
SOAPY_SDR_ONE_PACKET,
SOAPY_SDR_MORE_FRAGMENTS,
SOAPY_SDR_WAIT_TRIGGER,
SOAPY_SDR_TIMEOUT,
SOAPY_SDR_STREAM_ERROR,
SOAPY_SDR_CORRUPTION,
SOAPY_SDR_OVERFLOW,
SOAPY_SDR_NOT_SUPPORTED,
SOAPY_SDR_TIME_ERROR,
SOAPY_SDR_UNDERFLOW
end
| SoapySDR | https://github.com/JuliaTelecom/SoapySDR.jl.git |
|
[
"MIT",
"BSL-1.0"
] | 0.5.0 | e96812c38a7ca1c013d35c9515f05a1d023e2191 | code | 733 |
"""
error_to_string(error)
Calls `SoapySDR_errToStr(error)`, returning the string.
"""
function error_to_string(error)
ptr = SoapySDR_errToStr(error)
ptr === C_NULL ? "" : unsafe_string(ptr)
end
struct SoapySDRDeviceError <: Exception
status::Int
msg::String
end
function get_SoapySDRDeviceError()
return SoapySDRDeviceError(
SoapySDRDevice_lastStatus(),
unsafe_string(SoapySDRDevice_lastError()),
)
end
function with_error_check(f::Function)
val = f()
if SoapySDRDevice_lastStatus() != 0
throw(get_SoapySDRDeviceError())
end
return val
end
macro soapy_checked(ex)
return quote
with_error_check() do
$(esc(ex))
end
end
end
| SoapySDR | https://github.com/JuliaTelecom/SoapySDR.jl.git |
|
[
"MIT",
"BSL-1.0"
] | 0.5.0 | e96812c38a7ca1c013d35c9515f05a1d023e2191 | code | 6136 |
function SoapySDRDevice_listSensors(device)
len = Ref{Csize_t}()
args = SoapySDRDevice_listSensors(device, len)
(args, len[])
end
function SoapySDRDevice_getSettingInfo(device)
len = Ref{Csize_t}()
args = SoapySDRDevice_getSettingInfo(device, len)
(args, len[])
end
function SoapySDRDevice_listTimeSources(device)
len = Ref{Csize_t}()
args = SoapySDRDevice_listTimeSources(device, len)
(args, len[])
end
function SoapySDRDevice_listClockSources(device)
len = Ref{Csize_t}()
args = SoapySDRDevice_listClockSources(device, len)
(args, len[])
end
function SoapySDRDevice_listRegisterInterfaces(device)
len = Ref{Csize_t}()
args = SoapySDRDevice_listRegisterInterfaces(device, len)
(args, len[])
end
function SoapySDRDevice_listGPIOBanks(device)
len = Ref{Csize_t}()
args = SoapySDRDevice_listGPIOBanks(device, len)
(args, len[])
end
function SoapySDRDevice_listUARTs(device)
len = Ref{Csize_t}()
args = SoapySDRDevice_listUARTs(device, len)
(args, len[])
end
function SoapySDRDevice_listAntennas(device, direction, channel)
len = Ref{Csize_t}()
args = SoapySDRDevice_listAntennas(device, direction, channel, len)
(args, len[])
end
function SoapySDRDevice_getBandwidthRange(device, direction, channel)
len = Ref{Csize_t}()
args = SoapySDRDevice_getBandwidthRange(device, direction, channel, len)
(args, len[])
end
function SoapySDRDevice_getFrequencyRange(device, direction, channel)
len = Ref{Csize_t}()
args = SoapySDRDevice_getFrequencyRange(device, direction, channel, len)
(args, len[])
end
function SoapySDRDevice_listFrequencies(device, direction, channel)
len = Ref{Csize_t}()
args = SoapySDRDevice_listFrequencies(device, direction, channel, len)
(args, len[])
end
function SoapySDRDevice_getFrequencyRangeComponent(device, direction, channel, name)
len = Ref{Csize_t}()
args = SoapySDRDevice_getFrequencyRangeComponent(device, direction, channel, name, len)
(args, len[])
end
function SoapySDRDevice_listGains(device, direction, channel)
len = Ref{Csize_t}()
args = SoapySDRDevice_listGains(device, direction, channel, len)
(args, len[])
end
function SoapySDRDevice_getStreamFormats(device, direction, channel)
len = Ref{Csize_t}()
args = SoapySDRDevice_getStreamFormats(device, direction, channel, len)
(args, len[])
end
function SoapySDRDevice_getNativeStreamFormat(device, direction, channel)
fullscale = Ref{Cdouble}()
str = SoapySDRDevice_getNativeStreamFormat(device, direction, channel, fullscale)
(str, fullscale[])
end
function SoapySDRDevice_getSampleRateRange(device, direction, channel)
len = Ref{Csize_t}()
args = SoapySDRDevice_getSampleRateRange(device, direction, channel, len)
(args, len[])
end
function SoapySDRDevice_acquireReadBuffer(device::Device, stream, buffs, timeoutUs = 100000)
#SOAPY_SDR_API int SoapySDRDevice_acquireReadBuffer(SoapySDRDevice *device,
# SoapySDRStream *stream,
# size_t *handle,
# const void **buffs,
# int *flags,
# long long *timeNs,
# const long timeoutUs);
handle = Ref{Csize_t}()
flags = Ref{Cint}(0)
timeNs = Ref{Clonglong}(-1)
if !isopen(stream)
throw(InvalidStateException("stream is closed!", :closed))
end
bytes = SoapySDRDevice_acquireReadBuffer(
device,
stream,
handle,
buffs,
flags,
timeNs,
timeoutUs,
)
bytes, handle[], flags[], timeNs[]
end
function SoapySDRDevice_acquireWriteBuffer(
device::Device,
stream,
buffs,
timeoutUs = 100000,
)
#SOAPY_SDR_API int SoapySDRDevice_acquireWriteBuffer(SoapySDRDevice *device,
# SoapySDRStream *stream,
# size_t *handle,
# void **buffs,
# const long timeoutUs);
handle = Ref{Csize_t}()
if !isopen(stream)
throw(InvalidStateException("stream is closed!", :closed))
end
bytes = SoapySDRDevice_acquireWriteBuffer(device, stream, handle, buffs, timeoutUs)
return bytes, handle[]
end
function SoapySDRDevice_releaseWriteBuffer(
device::Device,
stream,
handle,
numElems,
flags = Ref{Cint}(0),
timeNs = 0,
)
#SOAPY_SDR_API void SoapySDRDevice_releaseWriteBuffer(SoapySDRDevice *device,
# SoapySDRStream *stream,
# const size_t handle,
# const size_t numElems,
# int *flags,
# const long long timeNs);
ccall(
(:SoapySDRDevice_releaseWriteBuffer, lib),
Cvoid,
(Ptr{SoapySDRDevice}, Ptr{SoapySDRStream}, Csize_t, Csize_t, Ref{Cint}, Clonglong),
device,
stream,
handle,
numElems,
flags,
timeNs,
)
flags[]
end
function SoapySDRDevice_readStream(device::Device, stream, buffs, numElems, timeoutUs)
if !isopen(stream)
throw(InvalidStateException("stream is closed!", :closed))
end
flags = Ref{Cint}()
timeNs = Ref{Clonglong}()
nelems = ccall(
(:SoapySDRDevice_readStream, lib),
Cint,
(
Ptr{SoapySDRDevice},
Ptr{SoapySDRStream},
Ptr{Cvoid},
Csize_t,
Ptr{Cint},
Ptr{Clonglong},
Clong,
),
device,
stream,
buffs,
numElems,
flags,
timeNs,
timeoutUs,
)
nelems, flags[], timeNs[]
end
function SoapySDRDevice_writeStream(
device::Device,
stream,
buffs,
numElems,
flags,
timeNs,
timeoutUs,
)
if !isopen(stream)
throw(InvalidStateException("stream is closed!", :closed))
end
flags = Ref{Cint}(flags)
nelems = ccall(
(:SoapySDRDevice_writeStream, lib),
Cint,
(
Ptr{SoapySDRDevice},
Ptr{SoapySDRStream},
Ptr{Cvoid},
Csize_t,
Ptr{Cint},
Clonglong,
Clong,
),
device,
stream,
buffs,
numElems,
flags,
timeNs,
timeoutUs,
)
nelems, flags[]
end
| SoapySDR | https://github.com/JuliaTelecom/SoapySDR.jl.git |
|
[
"MIT",
"BSL-1.0"
] | 0.5.0 | e96812c38a7ca1c013d35c9515f05a1d023e2191 | code | 34835 | # High level API exports
export Devices, Device, dB, gainrange
"""
Devices()
Device(args...)
Enumerates all detectable SDR devices on the system.
Indexing into the returned `Devices` object returns a list of
keywords used to create a `Device` struct.
Optionally pass in a list of keywords to filter the returned list.
Example:
```
Devices(driver="rtlsdr")
```
"""
struct Devices
kwargslist::KWArgsList
function Devices(args)
len = Ref{Csize_t}()
kwargs = SoapySDRDevice_enumerate(isnothing(args) ? C_NULL : args, len)
kwargs = KWArgsList(kwargs, len[])
if isempty(kwargs)
@warn "No devices available! Make sure a supported SDR module is included."
end
new(kwargs)
end
end
Base.length(d::Devices) = length(d.kwargslist)
function Devices(; kwargs...)
Devices(KWArgs(kwargs))
end
function Base.show(io::IO, d::Devices)
if length(d) == 0
println(io, "No devices available! Make sure a supported SDR module is included.")
end
for (i, dev) in enumerate(d.kwargslist)
print(io, "[$i] ")
join(io, dev, ", ")
println(io)
end
end
Base.getindex(d::Devices, i::Integer) = d.kwargslist[i]
Base.iterate(d::Devices, state = 1) = state > length(d) ? nothing : (d[state], state + 1)
####################################################################################################
# Device
####################################################################################################
"""
Device
A device is a collection of SDR channels, obtained from the `Devices()` list.
Fields:
- `info`
- `driver`
- `hardware`
- `tx`
- `rx`
- `sensors`
- `time_source`
- `time_sources`
- `clock_source`
- `clock_sources`
- `frontendmapping_rx`
- `frontendmapping_tx`
"""
mutable struct Device
ptr::Ptr{SoapySDRDevice}
function Device(args::KWArgs)
dev_ptr = SoapySDRDevice_make(args)
if dev_ptr == C_NULL
throw(ArgumentError("Unable to open device!"))
end
this = new(dev_ptr)
finalizer(this) do this
this.ptr != C_NULL && SoapySDRDevice_unmake(this.ptr)
this.ptr = Ptr{SoapySDRDevice}(C_NULL)
end
return this
end
end
function Device(f::Function, args::KWArgs)
dev = Device(args)
try
f(dev)
finally
finalize(dev)
end
end
Base.cconvert(::Type{<:Ptr{SoapySDRDevice}}, d::Device) = d
Base.unsafe_convert(::Type{<:Ptr{SoapySDRDevice}}, d::Device) = d.ptr
Base.isopen(d::Device) = d.ptr != C_NULL
function Base.show(io::IO, d::Device)
println(io, "SoapySDR ", d.hardware, " device")
println(io, " driver: ", d.driver)
println(io, " number of TX channels:", length(d.tx))
println(io, " number of RX channels:", length(d.rx))
println(io, " sensors: ", d.sensors)
println(io, " time_source: ", d.time_source)
println(io, " time_sources:", d.time_sources)
println(io, " clock_source: ", d.clock_source)
println(io, " clock_sources:", d.clock_sources)
println(io, " frontendmapping_rx: ", d.frontendmapping_rx)
println(io, " frontendmapping_tx: ", d.frontendmapping_tx)
println(io, " uarts: ", d.uarts)
println(io, " gpios: ", d.gpios)
println(io, " registers: ", d.registers)
print(io, " master_clock_rate: ")
print_unit(io, d.master_clock_rate)
end
function Base.getproperty(d::Device, s::Symbol)
if s === :info
KWArgs(SoapySDRDevice_getHardwareInfo(d))
elseif s === :driver
Symbol(unsafe_string(SoapySDRDevice_getDriverKey(d)))
elseif s === :hardware
Symbol(unsafe_string(SoapySDRDevice_getHardwareKey(d)))
elseif s === :tx
ChannelList(d, Tx)
elseif s === :rx
ChannelList(d, Rx)
elseif s === :sensors
ComponentList(SensorComponent, d)
elseif s === :time_sources
ComponentList(TimeSource, d)
elseif s === :uarts
ComponentList(UART, d)
elseif s === :registers
ComponentList(Register, d)
elseif s === :gpios
ComponentList(GPIO, d)
elseif s === :time_source
TimeSource(Symbol(unsafe_string(SoapySDRDevice_getTimeSource(d.ptr))))
elseif s === :clock_sources
ComponentList(ClockSource, d)
elseif s === :clock_source
ClockSource(Symbol(unsafe_string(SoapySDRDevice_getClockSource(d.ptr))))
elseif s === :frontendmapping_tx
unsafe_string(SoapySDRDevice_getFrontendMapping(d, Tx))
elseif s === :frontendmapping_rx
unsafe_string(SoapySDRDevice_getFrontendMapping(d, Rx))
elseif s === :master_clock_rate
SoapySDRDevice_getMasterClockRate(d.ptr) * u"Hz"
else
getfield(d, s)
end
end
function Base.setproperty!(c::Device, s::Symbol, v)
if s === :frontendmapping_tx
SoapySDRDevice_setFrontendMapping(c.ptr, Tx, v)
elseif s === :frontendmapping_rx
SoapySDRDevice_setFrontendMapping(c.ptr, Rx, v)
elseif s === :time_source
SoapySDRDevice_setTimeSource(c.ptr, string(v))
elseif s === :clock_source
SoapySDRDevice_setClockSource(c.ptr, string(v))
elseif s === :master_clock_rate
SoapySDRDevice_setMasterClockRate(c.ptr, v)
else
return setfield!(c, s, v)
end
end
function Base.propertynames(::Device)
return (
:ptr,
:info,
:driver,
:hardware,
:tx,
:rx,
:sensors,
:time_source,
:timesources,
:clock_source,
:clock_source,
:frontendmapping_rx,
:frontendmapping_tx,
:uarts,
:registers,
:gpios,
:master_clock_rate,
)
end
####################################################################################################
# Channel
####################################################################################################
"""
Channel
A channel on the given `Device`.
Note!!: A Channel can be created from a `Device` or extracted from a `ChannelList`. It should rarely
be necessary to create a Channel directly.
Has the following properties:
- `device::Device` - `Device` to which the `Channel` belongs
- `direction` - either `Tx` or `Rx`
- `idx` - channel index used by Soapy
- `info` - channel info consiting of `KWArgs`
- `antenna` - antenna name
- `gain_mode` - Automatic Gain control, `true`, `false`, or `missing`
- `gain_elements` - list of `GainElements` of the channel
- `gain` - effective gain, distributed amongst the `GainElements`
- `dc_offset_mode` - Automatic DC offset mode, `true`, `false` or `missing`
- `dc_offset` - DC offset value
- `iq_balance_mode` - Automatic IQ balance mode, `true`, `false` or `missing`
- `iq_balance` - IQ balance value
- `frequency_correction` - frequency correction value
- `sample_rate` - sample rate
- `bandwidth` - bandwidth
- `frequency` - center frequency
- `fullduplex` - full duplex mode with other (TX/RX) channels
- `native_stream_format` - native stream format
- `stream_formats` - supported stream formats (converted by Soapy)
- `fullscale` - full scale value
- `sensors` - sensor list
## Reading and writing to Components
gains, antennas, and sensors may consist of a chain or selectable subcomponets.
To set or read e.g. a sensors, one may use the following syntax:
dev = Devices()[1]
cr = dev.rx[1]
# read a sensor value
s1 = cr.sensors[1]
cr[s1]
# read and set the gain element
g1 = cr.gain_elements[1]
cr[g1]
cr[g1] = 4*u"dB"
# read and set the frequency component
f1 = cr.frequency_components[1]
cr[f1]
cr[f1] = 2.498*u"GHz"
"""
struct Channel
device::Device
direction::Direction
idx::Int
end
function Base.show(io::IO, c::Channel)
println(io, " antenna: ", c.antenna)
println(io, " antennas: ", c.antennas)
print(io, " bandwidth [ ")
join(io, map(x -> sprint(print_hz_range, x), bandwidth_ranges(c)), ", ")
println(io, " ]: ", pick_freq_unit(c.bandwidth))
print(io, " frequency [ ")
join(io, map(x -> sprint(print_hz_range, x), frequency_ranges(c)), ", ")
println(io, " ]: ", pick_freq_unit(c.frequency))
for element in FrequencyComponentList(c)
print(io, " ", element, " [ ")
join(io, map(x -> sprint(print_hz_range, x), frequency_ranges(c, element)), ", ")
println(io, " ]: ", pick_freq_unit(c[element]))
end
println(io, " gain_mode (AGC=true/false/missing): ", c.gain_mode)
println(io, " gain: ", c.gain)
println(io, " gain_elements:")
for element in c.gain_elements
println(io, " ", element, " [", gain_range(c, element), "]: ", c[element])
end
println(io, " fullduplex: ", c.fullduplex)
println(io, " stream_formats: ", c.stream_formats)
println(io, " native_stream_format: ", c.native_stream_format)
println(io, " fullscale: ", c.fullscale)
println(io, " sensors: ", c.sensors)
print(io, " sample_rate [ ")
join(io, map(x -> sprint(print_hz_range, x), sample_rate_ranges(c)), ", ")
println(io, " ]: ", pick_freq_unit(c.sample_rate))
println(io, " dc_offset_mode (true/false/missing): ", c.dc_offset_mode)
println(io, " dc_offset: ", c.dc_offset)
println(io, " iq_balance_mode (true/false/missing): ", c.iq_balance_mode)
println(io, " iq_balance: ", c.iq_balance)
fc = c.frequency_correction
println(io, " frequency_correction: ", fc, ismissing(fc) ? "" : " ppm")
end
"""
ChannelList
A grouping of channels on the Device.
Note: This should not be called directly, but rather through the Device.rx and Device.tx properties.
"""
struct ChannelList <: AbstractVector{Channel}
device::Device
direction::Direction
end
function Base.size(cl::ChannelList)
(SoapySDRDevice_getNumChannels(cl.device, cl.direction),)
end
function Base.getindex(cl::ChannelList, i::Integer)
checkbounds(cl, i)
Channel(cl.device, cl.direction, i - 1)
end
function Base.getproperty(c::Channel, s::Symbol)
if s === :info
return KWArgs(SoapySDRDevice_getChannelInfo(c.device.ptr, c.direction, c.idx))
elseif s === :antenna
ant = SoapySDRDevice_getAntenna(c.device.ptr, c.direction, c.idx)
return Antenna(Symbol(ant == C_NULL ? "" : unsafe_string(ant)))
elseif s === :antennas
return AntennaList(c)
elseif s === :gain
return SoapySDRDevice_getGain(c.device.ptr, c.direction, c.idx) * dB
elseif s === :gain_elements
return GainElementList(c)
elseif s === :dc_offset_mode
if !SoapySDRDevice_hasDCOffsetMode(c.device.ptr, c.direction, c.idx)
return missing
end
return SoapySDRDevice_getDCOffsetMode(c.device.ptr, c.direction, c.idx)
elseif s === :dc_offset
if !SoapySDRDevice_hasDCOffset(c.device.ptr, c.direction, c.idx)
return missing
end
i = Ref{Cdouble}(0)
q = Ref{Cdouble}(0)
SoapySDRDevice_getDCOffset(c.device.ptr, c.direction, c.idx, i, q)
return i[], q[]
elseif s === :iq_balance_mode
if !SoapySDRDevice_hasIQBalanceMode(c.device.ptr, c.direction, c.idx)
return missing
end
return SoapySDRDevice_getIQBalanceMode(c.device.ptr, c.direction, c.idx)
elseif s === :iq_balance
if !SoapySDRDevice_hasIQBalance(c.device.ptr, c.direction, c.idx)
return missing
end
i = Ref{Cdouble}(0)
q = Ref{Cdouble}(0)
SoapySDRDevice_getIQBalance(c.device.ptr, c.direction, c.idx, i, q)
return Complex(i[], q[])
elseif s === :gain_mode
if !SoapySDRDevice_hasGainMode(c.device.ptr, c.direction, c.idx)
return missing
end
return SoapySDRDevice_getGainMode(c.device.ptr, c.direction, c.idx)
elseif s === :frequency_correction
if !SoapySDRDevice_hasFrequencyCorrection(c.device.ptr, c.direction, c.idx)
return missing
end
# TODO: ppm unit?
return SoapySDRDevice_getFrequencyCorrection(c.device.ptr, c.direction, c.idx)
elseif s === :sample_rate
return SoapySDRDevice_getSampleRate(c.device.ptr, c.direction, c.idx) * Hz
elseif s === :sensors
ComponentList(SensorComponent, c.device, c)
elseif s === :bandwidth
return SoapySDRDevice_getBandwidth(c.device.ptr, c.direction, c.idx) * Hz
elseif s === :frequency
return SoapySDRDevice_getFrequency(c.device.ptr, c.direction, c.idx) * Hz
elseif s === :fullduplex
return Bool(SoapySDRDevice_getFullDuplex(c.device.ptr, c.direction, c.idx))
elseif s === :stream_formats
slist =
StringList(SoapySDRDevice_getStreamFormats(c.device.ptr, c.direction, c.idx)...)
return map(_stream_map_soapy2jl, slist)
elseif s === :native_stream_format
fmt, _ = SoapySDRDevice_getNativeStreamFormat(c.device.ptr, c.direction, c.idx)
return _stream_map_soapy2jl(fmt == C_NULL ? "" : unsafe_string(fmt))
elseif s === :fullscale
_, fullscale =
SoapySDRDevice_getNativeStreamFormat(c.device.ptr, c.direction, c.idx)
return fullscale
elseif s === :frequency_components
return FrequencyComponentList(c)
else
return getfield(c, s)
end
end
function Base.propertynames(::SoapySDR.Channel)
return (
:device,
:direction,
:idx,
:info,
:antenna,
:antennas,
:gain_elements,
:gain,
:dc_offset_mode,
:dc_offset,
:iq_balance_mode,
:iq_balance,
:gain_mode,
:frequency_correction,
:frequency_components,
:sample_rate,
:bandwidth,
:frequency,
:fullduplex,
:native_stream_format,
:stream_formats,
:fullscale,
:sensors,
)
end
function Base.setproperty!(c::Channel, s::Symbol, v)
if s === :antenna
if v isa Antenna
SoapySDRDevice_setAntenna(c.device.ptr, c.direction, c.idx, v.name)
elseif v isa Symbol || v isa String
SoapySDRDevice_setAntenna(c.device.ptr, c.direction, c.idx, v)
else
throw(ArgumentError("antenna must be an Antenna or a Symbol"))
end
elseif s === :frequency
if isa(v, Quantity)
SoapySDRDevice_setFrequency(
c.device.ptr,
c.direction,
c.idx,
uconvert(u"Hz", v).val,
C_NULL,
)
elseif isa(v, FreqSpec)
throw(ArgumentError("FreqSpec unsupported"))
else
throw(
ArgumentError(
"Frequency must be specified as either a Quantity or a FreqSpec!",
),
)
end
elseif s === :bandwidth
SoapySDRDevice_setBandwidth(
c.device.ptr,
c.direction,
c.idx,
uconvert(u"Hz", v).val,
)
elseif s === :gain_mode
SoapySDRDevice_setGainMode(c.device.ptr, c.direction, c.idx, v)
elseif s === :gain
SoapySDRDevice_setGain(c.device.ptr, c.direction, c.idx, uconvert(u"dB", v).val)
elseif s === :sample_rate
SoapySDRDevice_setSampleRate(
c.device.ptr,
c.direction,
c.idx,
uconvert(u"Hz", v).val,
)
elseif s === :dc_offset_mode
SoapySDRDevice_setDCOffsetMode(c.device.ptr, c.direction, c.idx, v)
elseif s === :iq_balance
SoapySDRDevice_setIQBalance(c.device.ptr, c.direction, c.idx, real(v), imag(v))
else
throw(ArgumentError("Channel has no property: $s"))
end
end
####################################################################################################
# Components
####################################################################################################
# Components is an internal mechaism to allow for dispatch and interface through the Julia API
# For example there may be several GainElements we list in a Channel. A Julian idiom for this is
# the set/getindex class of functions.
abstract type AbstractComponent end
Base.print(io::IO, c::AbstractComponent) = print(io, c.name)
Base.convert(::Type{T}, s::Symbol) where {T<:AbstractComponent} = T(s)
Base.convert(::Type{T}, s::String) where {T<:AbstractComponent} = T(Symbol(s))
Base.convert(::Type{Cstring}, s::AbstractComponent) =
Cstring(unsafe_convert(Ptr{UInt8}, s.name))
for e in (
:TimeSource,
:ClockSource,
:GainElement,
:Antenna,
:FrequencyComponent,
:SensorComponent,
:Setting,
:Register,
:UART,
:GPIO,
)
@eval begin
struct $e <: AbstractComponent
name::Symbol
end
function $e(s::AbstractString)
$e(Symbol(s))
end
end
end
struct ComponentList{T<:AbstractComponent} <: AbstractVector{T}
s::StringList
end
Base.size(l::ComponentList) = (length(l.s),)
Base.getindex(l::ComponentList{T}, i::Integer) where {T} = T(Symbol(l.s[i]))
function Base.show(io::IO, l::ComponentList{T}) where {T}
print(io, T, "[")
for c in l
print(io, c, ",")
end
print(io, "]")
end
for (e, f) in zip(
(:Antenna, :GainElement, :FrequencyComponent, :SensorComponent),
(
:SoapySDRDevice_listAntennas,
:SoapySDRDevice_listGains,
:SoapySDRDevice_listFrequencies,
:SoapySDRDevice_listSensors,
),
)
@eval begin
$f(channel::Channel) = $f(channel.device, channel.direction, channel.idx)
$(Symbol(string(e, "List")))(channel::Channel) =
ComponentList{$e}(StringList($f(channel)...))
end
end
function ComponentList(::Type{T}, d::Device) where {T<:AbstractComponent}
if T <: SensorComponent
ComponentList{SensorComponent}(StringList(SoapySDRDevice_listSensors(d.ptr)...))
elseif T <: TimeSource
ComponentList{TimeSource}(StringList(SoapySDRDevice_listTimeSources(d.ptr)...))
elseif T <: ClockSource
ComponentList{ClockSource}(StringList(SoapySDRDevice_listClockSources(d.ptr)...))
elseif T <: UART
ComponentList{UART}(StringList(SoapySDRDevice_listUARTs(d.ptr)...))
elseif T <: GPIO
ComponentList{GPIO}(StringList(SoapySDRDevice_listGPIOBanks(d.ptr)...))
elseif T <: Register
ComponentList{Register}(StringList(SoapySDRDevice_listRegisterInterfaces(d.ptr)...))
end
end
function ComponentList(::Type{T}, d::Device, c::Channel) where {T<:AbstractComponent}
len = Ref{Csize_t}()
if T <: SensorComponent
s = SoapySDRDevice_listChannelSensors(d.ptr, c.direction, c.idx, len)
ComponentList{SensorComponent}(StringList(s, len[]))
end
end
using Base: unsafe_convert
function Base.getindex(c::Channel, ge::GainElement)
SoapySDRDevice_getGainElement(c.device, c.direction, c.idx, ge.name) * dB
end
function Base.getindex(c::Channel, fe::FrequencyComponent)
SoapySDRDevice_getFrequencyComponent(c.device, c.direction, c.idx, fe.name) * Hz
end
function Base.getindex(c::Channel, se::SensorComponent)
unsafe_string(SoapySDRDevice_readChannelSensor(c.device, c.direction, c.idx, se.name))
end
function Base.getindex(c::Channel, se::Setting)
unsafe_string(SoapySDRDevice_readChannelSetting(c.device, c.direction, c.idx, se.name))
end
function Base.getindex(d::Device, se::SensorComponent)
unsafe_string(SoapySDRDevice_readSensor(d.ptr, se.name))
end
function Base.getindex(d::Device, se::Setting)
unsafe_string(SoapySDRDevice_readSetting(d.ptr, se.name))
end
function Base.getindex(d::Device, se::Tuple{Register,<:Integer})
SoapySDRDevice_readRegister(d.ptr, se[1].name, se[2])
end
function Base.setindex!(c::Channel, gain, ge::GainElement)
SoapySDRDevice_setGainElement(c.device, c.direction, c.idx, ge.name, gain.val)
return gain
end
function Base.setindex!(c::Channel, frequency, ge::FrequencyComponent)
SoapySDRDevice_setFrequencyComponent(
c.device,
c.direction,
c.idx,
ge.name,
uconvert(u"Hz", frequency).val,
C_NULL,
)
return frequency
end
function Base.setindex!(d::Device, v::AbstractString, ge::Setting)
SoapySDRDevice_writeSetting(d, ge.name, v)
return v
end
function Base.setindex!(c::Channel, v::AbstractString, se::Setting)
SoapySDRDevice_writeChannelSetting(c.device, c.direction, c.idx, se.name, v)
end
"""
Set a register value on a device:
```
dev[SoapySDR.Register("LMS7002M")] = (0x1234, 0x5678) # tuple of: (addr, value)
dev[(SoapySDR.Register("LMS7002M"), 0x1234)] = 0x5678 # this is also equivalent, and symmetric to the getindex form to read
```
"""
function Base.setindex!(d::Device, val::Tuple{<:Integer,<:Integer}, se::Register)
SoapySDRDevice_writeRegister(d.ptr, se.name, val[1], val[2])
end
function Base.setindex!(d::Device, val::Integer, se::Tuple{Register,<:Integer})
SoapySDRDevice_writeRegister(d.ptr, se[1].name, se[2], val)
end
## GainElement
function _gainrange(soapyr::SoapySDRRange)
if soapyr.step == 0.0
# Represents an interval rather than a range
return (soapyr.minimum * dB) .. (soapyr.maximum * dB)
end
# TODO
@warn "Step ranges are not supported for gain elements. Returning Interval instead. Step: $(soapyr.step*dB)"
#return range(soapyr.minimum*dB; stop=soapyr.maximum*dB, step=soapyr.step*dB)
return (soapyr.minimum * dB) .. (soapyr.maximum * dB)
end
function gainrange(c::Channel)
return _gainrange(SoapySDRDevice_getGainRange(c.device, c.direction, c.idx))
end
gainrange(c::Channel, ge::GainElement) = return _gainrange(
SoapySDRDevice_getGainElementRange(c.device, c.direction, c.idx, ge.name),
)
function _hzrange(soapyr::SoapySDRRange)
if soapyr.step == 0.0
# Represents an interval rather than a range
return (soapyr.minimum * Hz) .. (soapyr.maximum * Hz)
end
return range(soapyr.minimum * Hz; stop = soapyr.maximum * Hz, step = soapyr.step * Hz)
end
function bandwidth_ranges(c::Channel)
(ptr, len) = SoapySDRDevice_getBandwidthRange(c.device.ptr, c.direction, c.idx)
ptr == C_NULL && return SoapySDRRange[]
arr = map(_hzrange, unsafe_wrap(Array, Ptr{SoapySDRRange}(ptr), (len,)))
SoapySDR_free(ptr)
arr
end
function frequency_ranges(c::Channel)
(ptr, len) = SoapySDRDevice_getFrequencyRange(c.device.ptr, c.direction, c.idx)
ptr == C_NULL && return SoapySDRRange[]
arr = map(_hzrange, unsafe_wrap(Array, Ptr{SoapySDRRange}(ptr), (len,)))
SoapySDR_free(ptr)
arr
end
function frequency_ranges(c::Channel, fe::FrequencyComponent)
(ptr, len) =
SoapySDRDevice_getFrequencyRangeComponent(c.device.ptr, c.direction, c.idx, fe.name)
ptr == C_NULL && return SoapySDRRange[]
arr = map(_hzrange, unsafe_wrap(Array, Ptr{SoapySDRRange}(ptr), (len,)))
SoapySDR_free(ptr)
arr
end
function gain_range(c::Channel, ge::GainElement)
ptr = SoapySDRDevice_getGainElementRange(c.device.ptr, c.direction, c.idx, ge.name)
ptr
end
function sample_rate_ranges(c::Channel)
(ptr, len) = SoapySDRDevice_getSampleRateRange(c.device.ptr, c.direction, c.idx)
ptr == C_NULL && return SoapySDRRange[]
arr = map(_hzrange, unsafe_wrap(Array, Ptr{SoapySDRRange}(ptr), (len,)))
SoapySDR_free(ptr)
arr
end
"""
list_sample_rates(::Channel)
List the natively supported sample rates for a given channel.
"""
function list_sample_rates(c::Channel)
len = Ref{Csize_t}(0)
ptr = SoapySDRDevice_listSampleRates(c.device.ptr, c.direction, c.idx, len)
arr = unsafe_wrap(Array, Ptr{Float64}(ptr), (len[],)) * Hz
SoapySDR_free(ptr)
arr
end
### Frequency Setting
struct FreqSpec{T}
val::T
kwargs::Dict{Any,String}
end
####################################################################################################
# Stream
####################################################################################################
"""
SoapySDR.Stream(channels)
SoapySDR.Stream(::Type{T}, channels)
Constructs a `Stream{T}` where `T` is the stream type of the device. If unspecified,
the native format will be used.
Fields:
- nchannels - The number of channels in the stream
- mtu - The stream Maximum Transmission Unit
- num_direct_access_buffers - The numer of direct access buffers available in the stream
## Example
```
SoapySDR.Stream(Devices()[1].rx)
```
"""
mutable struct Stream{T}
d::Device
nchannels::Int
ptr::Ptr{SoapySDRStream}
function Stream{T}(d::Device, nchannels, ptr::Ptr{SoapySDRStream}) where {T}
this = new{T}(d, Int(nchannels), ptr)
finalizer(this) do obj
isopen(d) || return
SoapySDRDevice_closeStream(d, obj.ptr)
obj.ptr = Ptr{SoapySDRStream}(C_NULL)
end
return this
end
end
Base.cconvert(::Type{<:Ptr{SoapySDRStream}}, s::Stream) = s
Base.unsafe_convert(::Type{<:Ptr{SoapySDRStream}}, s::Stream) = s.ptr
Base.isopen(s::Stream) = s.ptr != C_NULL && isopen(s.d)
streamtype(::Stream{T}) where {T} = T
function Base.setproperty!(c::Stream, s::Symbol, v)
return setfield!(c, s, v)
end
function Base.propertynames(::Stream)
return (:d, :nchannels, :mtu, :num_direct_access_buffers)
end
function Base.getproperty(stream::Stream, s::Symbol)
if s === :mtu
if !isopen(stream)
throw(InvalidStateException("Stream is closed!", :closed))
end
SoapySDRDevice_getStreamMTU(stream.d.ptr, stream.ptr)
elseif s === :num_direct_access_buffers
if !isopen(stream)
throw(InvalidStateException("Stream is closed!", :closed))
end
SoapySDRDevice_getNumDirectAccessBuffers(stream.d.ptr, stream.ptr)
else
return getfield(stream, s)
end
end
function Base.show(io::IO, s::Stream)
print(io, "Stream on ", s.d.hardware)
end
function Stream(format::Type, device::Device, direction::Direction; kwargs...)
Stream{T}(
device,
1,
SoapySDRDevice_setupStream(
device,
direction,
string(format),
C_NULL,
0,
KWArgs(kwargs),
),
)
end
function Stream(format::Type, channels::AbstractVector{T}; kwargs...) where {T<:Channel}
soapy_format = _stream_map_jl2soapy(format)
isempty(channels) && error(
"Must specify at least one channel or use the device/direction constructor for automatic.",
)
device = first(channels).device
direction = first(channels).direction
if !all(channels) do channel
channel.device == device && channel.direction == direction
end
throw(ArgumentError("Channels must agree on device and direction"))
end
Stream{format}(
device,
length(channels),
SoapySDRDevice_setupStream(
device,
direction,
soapy_format,
map(x -> x.idx, channels),
length(channels),
KWArgs(kwargs),
),
)
end
function Stream(channels::AbstractVector{T}; kwargs...) where {T<:Channel}
native_format = promote_type(map(c -> c.native_stream_format, channels)...)
if native_format <: AbstractComplexInteger
@warn "$(string(native_format)) may be poorly supported, it is recommend to specify a different type with Stream(format::Type, channels)"
end
Stream(native_format, channels; kwargs...)
end
function Stream(format::Type, channel::Channel; kwargs...)
Stream(format, [channel], kwargs...)
end
function Stream(channel::Channel; kwargs...)
Stream([channel], kwargs...)
end
function Stream(f::Function, args...; kwargs...)
stream = Stream(args...; kwargs...)
try
f(stream)
finally
finalize(stream)
end
end
const SoapyStreamFlags = Dict(
"END_BURST" => SOAPY_SDR_END_BURST,
"HAS_TIME" => SOAPY_SDR_HAS_TIME,
"END_ABRUPT" => SOAPY_SDR_END_ABRUPT,
"ONE_PACKET" => SOAPY_SDR_ONE_PACKET,
"MORE_FRAGMENTS" => SOAPY_SDR_MORE_FRAGMENTS,
"WAIT_TRIGGER" => SOAPY_SDR_WAIT_TRIGGER,
)
function flags_to_set(flags)
s = Set{String}()
for (name, val) in SoapyStreamFlags
if val & flags != 0
push!(s, name)
end
end
return s
end
function Base.read!(
::Stream,
::NTuple;
kwargs...
)
error("Buffers should be a Vector of Vectors rather than NTuple.")
end
"""
read!(s::SoapySDR.Stream{T}, buffers::AbstractVector{AbstractVector{T}}; [timeout], [flags::Ref{Int}], [throw_error=false])
Read data from the device into the given buffers.
"""
function Base.read!(
s::Stream{T},
buffers::AbstractVector{<:AbstractVector{T}};
timeout = nothing,
flags::Union{Ref{Int},Nothing} = nothing,
throw_error::Bool = false,
) where {T}
t_start = time()
timeout === nothing && (timeout = 0.1u"s") # Default from SoapySDR upstream
timeout_s = uconvert(u"s", timeout).val
timeout_us = uconvert(u"μs", timeout).val
total_nread = 0
samples_to_read = length(first(buffers))
if !all(length(b) == samples_to_read for b in buffers)
throw(ArgumentError("Buffers must all be same length!"))
end
GC.@preserve buffers while total_nread < samples_to_read
# collect list of pointers to pass to SoapySDR
buff_ptrs = pointer(map(b -> pointer(b, total_nread + 1), buffers))
nread, out_flags, timens = SoapySDRDevice_readStream(
s.d,
s,
buff_ptrs,
samples_to_read - total_nread,
timeout_us,
)
if typeof(flags) <: Ref
flags[] |= out_flags
end
if nread < 0
if throw_error
throw(SoapySDRDeviceError(nread, error_to_string(nread)))
end
else
total_nread += nread
end
if time() > t_start + timeout_s
# We've timed out, return early and warn. Something is probably wrong.
@warn(
"readStream timeout!",
timeout = timeout_s,
total_nread,
samples_to_read,
flags = join(flags_to_set(out_flags), ","),
)
return buffers
end
end
return buffers
end
"""
read(s::SoapySDR.Stream{T}, nb::Integer; [timeout])
Read at most `nb` samples from s
"""
function Base.read(s::Stream{T}, n::Integer; timeout = nothing) where {T}
bufs = [Vector{T}(undef, n) for _ in 1:s.nchannels]
read!(s, bufs; timeout)
bufs
end
function activate!(s::Stream; flags = 0, timens = nothing, numElems = nothing)
if !isopen(s)
throw(InvalidStateException("stream is closed!", :closed))
end
if timens === nothing
timens = 0
else
timens = uconvert(u"ns", timens).val
end
if numElems === nothing
numElems = 0
end
SoapySDRDevice_activateStream(s.d, s, flags, timens, numElems)
return nothing
end
function activate!(f::Function, streams::AbstractVector{<:Stream}; kwargs...)
activated_streams = Vector{SoapySDR.Stream}(undef, length(streams))
try
for i in eachindex(streams)
s = streams[i]
activate!(s; kwargs...)
activated_streams[i] = s
end
f()
finally
for s in activated_streams
deactivate!(s; kwargs...)
end
end
end
function activate!(f::Function, s::Stream; kwargs...)
try
activate!(s; kwargs...)
f()
finally
deactivate!(s; kwargs...)
end
end
function deactivate!(s::Stream; flags = 0, timens = nothing)
SoapySDRDevice_deactivateStream(
s.d,
s,
flags,
timens === nothing ? 0 : uconvert(u"ns", timens).val,
)
return nothing
end
function Base.write(
::Stream,
::NTuple;
kwargs...
)
error("Buffers should be a Vector of Vectors rather than NTuple.")
end
"""
write(s::SoapySDR.Stream{T}, buffer::AbstractVector{AbstractVector{T}}; [timeout], [flags::Ref{Int}], [throw_error=false]) where {N, T}
Write data from the given buffers into the device. The buffers must all be the same length.
"""
function Base.write(
s::Stream{T},
buffers::AbstractVector{<:AbstractVector{T}};
timeout = nothing,
flags::Union{Ref{Int},Nothing} = nothing,
throw_error::Bool = false,
) where {T}
t_start = time()
timeout === nothing && (timeout = 0.1u"s") # Default from SoapySDR upstream
timeout_s = uconvert(u"s", timeout).val
timeout_us = uconvert(u"μs", timeout).val
total_nwritten = 0
samples_to_write = length(first(buffers))
if !all(length(b) == samples_to_write for b in buffers)
throw(ArgumentError("Buffers must all be same length!"))
end
if length(buffers) != s.nchannels
throw(ArgumentError("Must provide buffers for every channel in stream!"))
end
GC.@preserve buffers while total_nwritten < samples_to_write
buff_ptrs = pointer(map(b -> pointer(b, total_nwritten + 1), buffers))
nwritten, out_flags = SoapySDRDevice_writeStream(
s.d,
s,
buff_ptrs,
samples_to_write - total_nwritten,
0,
0,
timeout_us,
)
if typeof(flags) <: Ref
flags[] |= out_flags
end
if nwritten < 0
if throw_error
throw(SoapySDRDeviceError(nwritten, error_to_string(nwritten)))
end
else
total_nwritten += nwritten
end
if time() > t_start + timeout_s
# We've timed out, return early and warn. Something is probably wrong.
@warn(
"writeStream timeout!",
timeout = timeout_s,
total_nwritten,
samples_to_write,
flags = join(flags_to_set(out_flags), ","),
)
return buffers
end
end
return buffers
end
"""
get_sensor_info(::Device, ::String)
Read the sensor extracted from `list_sensors`.
Returns: the value as a string.
Note: Appropriate conversions need to be done by the user.
"""
function get_sensor_info(d::Device, name)
SoapySDRDevice_getSensorInfo(d.ptr, name)
end
## Time API
"""
has_hardware_time(::Device, what::String)
Query if the Device has hardware time for the given source.
"""
function has_hardware_time(d::Device, what::String)
SoapySDRDevice_hasHardwareTime(d.ptr, what)
end
"""
get_hardware_time(::Device, what::String)
Get hardware time for the given source.
"""
function get_hardware_time(d::Device, what::String)
SoapySDRDevice_getHardwareTime(d.ptr, what)
end
"""
set_hardware_time(::Device, timeNs::Int64 what::String)
Set hardware time for the given source.
"""
function set_hardware_time(d::Device, timeNs::Int64, what::String)
SoapySDRDevice_setHardwareTime(d.ptr, timeNs, what)
end
| SoapySDR | https://github.com/JuliaTelecom/SoapySDR.jl.git |
|
[
"MIT",
"BSL-1.0"
] | 0.5.0 | e96812c38a7ca1c013d35c9515f05a1d023e2191 | code | 2047 | """
Log handler to forward Soapy logs to Julia logs.
"""
function logger_soapy2jl(level, cmessage)
#SOAPY_SDR_FATAL = 1 #!< A fatal error. The application will most likely terminate. This is the highest priority.
#SOAPY_SDR_CRITICAL = 2 #!< A critical error. The application might not be able to continue running successfully.
#SOAPY_SDR_ERROR = 3 #!< An error. An operation did not complete successfully, but the application as a whole is not affected.
#SOAPY_SDR_WARNING = 4 #!< A warning. An operation completed with an unexpected result.
#SOAPY_SDR_NOTICE = 5 #!< A notice, which is an information with just a higher priority.
#SOAPY_SDR_INFO = 6 #!< An informational message, usually denoting the successful completion of an operation.
#SOAPY_SDR_DEBUG = 7 #!< A debugging message.
#SOAPY_SDR_TRACE = 8 #!< A tracing message. This is the lowest priority.
#SOAPY_SDR_SSI = 9 #!< Streaming status indicators such as "U" (underflow) and "O" (overflow).
message = unsafe_string(cmessage)
level = SoapySDRLogLevel(level)
if level in (SOAPY_SDR_FATAL, SOAPY_SDR_CRITICAL, SOAPY_SDR_ERROR)
@error message
elseif level == SOAPY_SDR_WARNING
@warn message
elseif level in (SOAPY_SDR_NOTICE, SOAPY_SDR_INFO)
@info message
elseif level in (SOAPY_SDR_DEBUG, SOAPY_SDR_TRACE, SOAPY_SDR_SSI)
@debug message
else
println("SoapySDR_jll: ", message)
end
end
"""
Initialize the log handler to convert Soapy logs to Julia logs.
This should be called once at the start of a script before doing work.
"""
function register_log_handler()
julia_log_handler = @cfunction(logger_soapy2jl, Cvoid, (Cint, Cstring))
SoapySDR_registerLogHandler(julia_log_handler)
end
"""
Set the log level threshold.
Log messages with lower priority are dropped.
NOTE: This uses SoapySDR log level number, which is different from Julia log level number.
"""
function set_log_level(level)
SoapySDR_setLogLevel(SoapySDRLogLevel(level))
end
| SoapySDR | https://github.com/JuliaTelecom/SoapySDR.jl.git |
|
[
"MIT",
"BSL-1.0"
] | 0.5.0 | e96812c38a7ca1c013d35c9515f05a1d023e2191 | code | 2341 | # Map of Soapy stream formats to Julia types
"""SOAPY_SDR_TX SOAPY_SDR_RX"""
@enum Direction Tx = 0 Rx = 1
"""
Abstract type denoting a Complex(U)Int(12/4) type.
These need to be specially handled with bit shifting, and
we do no data processing in this library, so this
exists for convience to other package developers.
Subtypes are `ComplexInt` and `ComplexUInt`,
for convience with handling sign extends.
"""
abstract type AbstractComplexInteger end
"""
Type indicating a Complex Int format.
Parameter `T` indicates the number of bits.
"""
struct ComplexInt{T} <: AbstractComplexInteger end
"""
Type indicating a Complex Unsigned Int format.
Parameter `T` indicates the number of bits.
"""
struct ComplexUInt{T} <: AbstractComplexInteger end
const _stream_type_pairs = [
(SOAPY_SDR_CF64, Complex{Float64}),
(SOAPY_SDR_CF32, Complex{Float32}),
(SOAPY_SDR_CS32, Complex{Int32}),
(SOAPY_SDR_CU32, Complex{UInt32}),
(SOAPY_SDR_CS16, Complex{Int16}),
(SOAPY_SDR_CU16, Complex{UInt16}),
(SOAPY_SDR_CS12, ComplexInt{12}),
(SOAPY_SDR_CU12, ComplexUInt{12}),
(SOAPY_SDR_CS8, Complex{Int8}),
(SOAPY_SDR_CU8, Complex{UInt8}),
(SOAPY_SDR_CS4, ComplexInt{4}),
(SOAPY_SDR_CU4, ComplexUInt{4}),
(SOAPY_SDR_F64, Float64),
(SOAPY_SDR_F32, Float32),
(SOAPY_SDR_S32, Int32),
(SOAPY_SDR_U32, UInt32),
(SOAPY_SDR_S16, Int16),
(SOAPY_SDR_U16, UInt16),
(SOAPY_SDR_S8, Int8),
(SOAPY_SDR_U8, UInt8),
("", Nothing),
]
"""
Type map from SoapySDR Stream formats to Julia types.
Note: Please see ComplexUInt and ComplexUInt if using 12 or 4 bit complex types.
"""
const _stream_type_soapy2jl = Dict{String,Type}(_stream_type_pairs)
"""
Type map from SoapySDR Stream formats to Julia types.
Note: Please see ComplexUInt and ComplexUInt if using 12 or 4 bit complex types.
"""
const _stream_type_jl2soapy = Dict{Type,String}(reverse.(_stream_type_pairs))
function _stream_map_jl2soapy(stream_type)
if !haskey(_stream_type_jl2soapy, stream_type)
error("Unsupported stream type: ", stream_type)
end
return _stream_type_jl2soapy[stream_type]
end
function _stream_map_soapy2jl(stream_type)
if !haskey(_stream_type_soapy2jl, stream_type)
error("Unsupported stream type: ", stream_type)
end
return _stream_type_soapy2jl[stream_type]
end
| SoapySDR | https://github.com/JuliaTelecom/SoapySDR.jl.git |
|
[
"MIT",
"BSL-1.0"
] | 0.5.0 | e96812c38a7ca1c013d35c9515f05a1d023e2191 | code | 4159 |
## KWArgs
export KWArgs
mutable struct KWArgs <: AbstractDict{String,String}
box::Base.RefValue{SoapySDRKwargs}
function KWArgs(kw::SoapySDRKwargs; owned::Bool = true)
this = new(Ref(kw))
owned && finalizer(SoapySDRKwargs_clear, this)
return this
end
end
KWArgs() = KWArgs(SoapySDRKwargs_fromString(""))
function KWArgs(kwargs::Base.Iterators.Pairs)
args = KWArgs()
for kv in kwargs
args[string(kv.first)] = kv.second
end
return args
end
Base.unsafe_convert(T::Type{Ptr{SoapySDRKwargs}}, args::KWArgs) =
Base.unsafe_convert(T, args.box)
Base.String(args::KWArgs) = unsafe_string(SoapySDRKwargs_toString(args))
Base.parse(::Type{KWArgs}, str::String) = KWArgs(SoapySDRKwargs_fromString(str))
function Base.show(io::IO, ::MIME{Symbol("text/plain")}, args::KWArgs)
print(io, "KWArgs(")
print(io, String(args))
print(io, ")")
end
function Base.getindex(args::KWArgs, key)
key = convert(String, key)
cstr = SoapySDRKwargs_get(args, key)
cstr == C_NULL && throw(KeyError(key))
unsafe_string(cstr)
end
function Base.setindex!(args::KWArgs, value, key)
key = convert(String, key)
value = convert(String, value)
SoapySDRKwargs_set(args, key, value)
args
end
Base.length(kw::KWArgs) = kw.box[].size
function Base.iterate(kw::KWArgs, i = 1)
i > length(kw) && return nothing
GC.@preserve kw begin
return (
unsafe_string(unsafe_load(kw.box[].keys, i)) =>
unsafe_string(unsafe_load(kw.box[].vals, i))
),
i + 1
end
end
## KWArgsList
mutable struct KWArgsList <: AbstractVector{KWArgs}
ptr::Ptr{SoapySDRKwargs}
length::Csize_t
function KWArgsList(ptr::Ptr{SoapySDRKwargs}, length::Csize_t)
this = new(ptr, length)
finalizer(this) do this
SoapySDRKwargsList_clear(this, this.length)
end
this
end
end
Base.size(kwl::KWArgsList) = (kwl.length,)
function Base.unsafe_convert(::Type{Ptr{SoapySDRKwargs}}, kwl::KWArgsList)
@assert kwl.ptr != C_NULL
kwl.ptr
end
function Base.getindex(kwl::KWArgsList, i::Integer)
@boundscheck checkbounds(kwl, i)
KWArgs(unsafe_load(kwl.ptr, i); owned = false)
end
## ArgInfoList
mutable struct ArgInfoList <: AbstractVector{SoapySDRArgInfo}
ptr::Ptr{SoapySDRArgInfo}
length::Csize_t
function ArgInfoList(ptr::Ptr{SoapySDRArgInfo}, length::Csize_t)
this = new(ptr, length)
finalizer(this) do this
SoapySDRArgInfoList_clear(this.ptr, this.length)
end
return this
end
end
Base.size(kwl::ArgInfoList) = (kwl.length,)
function Base.getindex(kwl::ArgInfoList, i::Integer)
@boundscheck checkbounds(kwl, i)
unsafe_load(kwl.ptr, i)
end
## StringList
mutable struct StringList <: AbstractVector{String}
strs::Ptr{Cstring}
length::Csize_t
function StringList(strs::Ptr{Cstring}, length::Integer; owned::Bool = true)
this = new(strs, Csize_t(length))
if owned
finalizer(SoapySDRStrings_clear, this)
end
this
end
end
function StringList(strs::Ptr{Ptr{Cchar}}, length::Integer; kwargs...)
StringList(reinterpret(Ptr{Cstring}, strs), length; kwargs...)
end
Base.size(s::StringList) = (s.length,)
function Base.getindex(s::StringList, i::Integer)
checkbounds(s, i)
unsafe_string(unsafe_load(s.strs, i))
end
SoapySDRStrings_clear(s::StringList) =
GC.@preserve s SoapySDRStrings_clear(pointer_from_objref(s), s.length)
## ArgInfo
function Base.show(io::IO, s::SoapySDRArgInfo)
println(io, "name: ", unsafe_string(s.name))
println(io, "key: ", unsafe_string(s.key))
#println(io, "value: ", unsafe_string(s.value))
println(io, "description: ", unsafe_string(s.description))
println(io, "units: ", unsafe_string(s.units))
#type
#range
println(io, "options: ", StringList(s.options, s.numOptions; owned = false))
println(io, "optionNames: ", StringList(s.optionNames, s.numOptions; owned = false))
end
function Base.show(io::IO, s::SoapySDRRange)
print(io, s.minimum, ":", s.step, ":", s.maximum)
end
| SoapySDR | https://github.com/JuliaTelecom/SoapySDR.jl.git |
|
[
"MIT",
"BSL-1.0"
] | 0.5.0 | e96812c38a7ca1c013d35c9515f05a1d023e2191 | code | 1829 | ####################################################################################################
# Unit Printing
####################################################################################################
# Express everything in (kHz, MHz, GHz)
function pick_freq_unit(val::Quantity)
iszero(val.val) && return val
abs(val) >= 1.0u"GHz" ? uconvert(u"GHz", val) :
abs(val) >= 1.0u"MHz" ? uconvert(u"MHz", val) : uconvert(u"kHz", val)
end
# Print to 3 digits of precision, no decimal point
print_3_digit(io::IO, val::Quantity) = print(io, Base.Ryu.writeshortest(
round(val.val, sigdigits = 3),
false, #= plus =#
false, #= space =#
false, #= hash =#
))
function print_unit(io::IO, val::Quantity)
print_3_digit(io, val)
print(io, " ", unit(val))
end
function print_unit_interval(io::IO, min, max)
if unit(min) == unit(max) || iszero(min.val)
print_3_digit(io, min)
print(io, "..")
print_3_digit(io, max)
print(io, " ", unit(max))
else
print_unit(io, min)
print(io, " .. ")
print_unit(io, max)
end
end
function print_unit_steprange(io::IO, min, max, step)
print_unit(io, min)
print(io, ":")
print_unit(io, step)
print(io, ":")
print_unit(io, max)
end
print_unit_interval(io::IO, x::Interval{<:Any,Closed,Closed}) =
print_unit_interval(io, minimum(x), maximum(x))
using Intervals: Closed
function print_hz_range(io::IO, x::Interval{<:Any,Closed,Closed})
min, max = pick_freq_unit(minimum(x)), pick_freq_unit(maximum(x))
print_unit_interval(io, min, max)
end
function print_hz_range(io::IO, x::AbstractRange)
min, step, max =
pick_freq_unit(first(x)), pick_freq_unit(Base.step(x)), pick_freq_unit(last(x))
print_unit_steprange(io, min, max, step)
end
| SoapySDR | https://github.com/JuliaTelecom/SoapySDR.jl.git |
|
[
"MIT",
"BSL-1.0"
] | 0.5.0 | e96812c38a7ca1c013d35c9515f05a1d023e2191 | code | 434 | """
versioninfo(io::IO=stdout)
Print information about the version of SoapySDR in use.
"""
function versioninfo(io = stdout; kwargs...)
api_ver = unsafe_string(SoapySDR_getAPIVersion())
abi_ver = unsafe_string(SoapySDR_getABIVersion())
lib_ver = unsafe_string(SoapySDR_getLibVersion())
println(io, "API Version ", api_ver)
println(io, "ABI Version ", abi_ver)
println(io, "Library Version ", lib_ver)
end
| SoapySDR | https://github.com/JuliaTelecom/SoapySDR.jl.git |
|
[
"MIT",
"BSL-1.0"
] | 0.5.0 | e96812c38a7ca1c013d35c9515f05a1d023e2191 | code | 104651 | # typedef void ( * SoapySDRConverterFunction ) ( const void * , void * , const size_t , const double )
"""
A typedef for declaring a ConverterFunction to be maintained in the ConverterRegistry.
A converter function copies and optionally converts an input buffer of one format into an
output buffer of another format.
The parameters are (input pointer, output pointer, number of elements, optional scalar)
"""
const SoapySDRConverterFunction = Ptr{Cvoid}
"""
SoapySDRConverterFunctionPriority
Allow selection of a converter function with a given source and target format.
"""
@cenum SoapySDRConverterFunctionPriority::UInt32 begin
SOAPY_SDR_CONVERTER_GENERIC = 0
SOAPY_SDR_CONVERTER_VECTORIZED = 3
SOAPY_SDR_CONVERTER_CUSTOM = 5
end
"""
SoapySDRConverter_listTargetFormats(sourceFormat, length)
Get a list of existing target formats to which we can convert the specified source from.
\\param sourceFormat the source format markup string
\\param [out] length the number of valid target formats
\\return a list of valid target formats
"""
function SoapySDRConverter_listTargetFormats(sourceFormat, length)
ccall(
(:SoapySDRConverter_listTargetFormats, soapysdr),
Ptr{Ptr{Cchar}},
(Ptr{Cchar}, Ptr{Csize_t}),
sourceFormat,
length,
)
end
"""
SoapySDRConverter_listSourceFormats(targetFormat, length)
Get a list of existing source formats to which we can convert the specified target from.
\\param targetFormat the target format markup string
\\param [out] length the number of valid source formats
\\return a list of valid source formats
"""
function SoapySDRConverter_listSourceFormats(targetFormat, length)
ccall(
(:SoapySDRConverter_listSourceFormats, soapysdr),
Ptr{Ptr{Cchar}},
(Ptr{Cchar}, Ptr{Csize_t}),
targetFormat,
length,
)
end
"""
SoapySDRConverter_listPriorities(sourceFormat, targetFormat, length)
Get a list of available converter priorities for a given source and target format.
\\param sourceFormat the source format markup string
\\param targetFormat the target format markup string
\\param [out] length the number of priorities
\\return a list of priorities or nullptr if none are found
"""
function SoapySDRConverter_listPriorities(sourceFormat, targetFormat, length)
ccall(
(:SoapySDRConverter_listPriorities, soapysdr),
Ptr{SoapySDRConverterFunctionPriority},
(Ptr{Cchar}, Ptr{Cchar}, Ptr{Csize_t}),
sourceFormat,
targetFormat,
length,
)
end
"""
SoapySDRConverter_getFunction(sourceFormat, targetFormat)
Get a converter between a source and target format with the highest available priority.
\\param sourceFormat the source format markup string
\\param targetFormat the target format markup string
\\return a conversion function pointer or nullptr if none are found
"""
function SoapySDRConverter_getFunction(sourceFormat, targetFormat)
ccall(
(:SoapySDRConverter_getFunction, soapysdr),
SoapySDRConverterFunction,
(Ptr{Cchar}, Ptr{Cchar}),
sourceFormat,
targetFormat,
)
end
"""
SoapySDRConverter_getFunctionWithPriority(sourceFormat, targetFormat, priority)
Get a converter between a source and target format with a given priority.
\\param sourceFormat the source format markup string
\\param targetFormat the target format markup string
\\return a conversion function pointer or nullptr if none are found
"""
function SoapySDRConverter_getFunctionWithPriority(sourceFormat, targetFormat, priority)
ccall(
(:SoapySDRConverter_getFunctionWithPriority, soapysdr),
SoapySDRConverterFunction,
(Ptr{Cchar}, Ptr{Cchar}, SoapySDRConverterFunctionPriority),
sourceFormat,
targetFormat,
priority,
)
end
"""
SoapySDRConverter_listAvailableSourceFormats(length)
Get a list of known source formats in the registry.
\\param [out] length the number of known source formats
\\return a list of known source formats
"""
function SoapySDRConverter_listAvailableSourceFormats(length)
ccall(
(:SoapySDRConverter_listAvailableSourceFormats, soapysdr),
Ptr{Ptr{Cchar}},
(Ptr{Csize_t},),
length,
)
end
mutable struct SoapySDRDevice end
mutable struct SoapySDRStream end
"""
SoapySDRDevice_lastStatus()
Get the last status code after a Device API call.
The status code is cleared on entry to each Device call.
When an device API call throws, the C bindings catch
the exception, and set a non-zero last status code.
Use lastStatus() to determine success/failure for
Device calls without integer status return codes.
"""
function SoapySDRDevice_lastStatus()
ccall((:SoapySDRDevice_lastStatus, soapysdr), Cint, ())
end
"""
SoapySDRDevice_lastError()
Get the last error message after a device call fails.
When an device API call throws, the C bindings catch
the exception, store its message in thread-safe storage,
and return a non-zero status code to indicate failure.
Use lastError() to access the exception's error message.
"""
function SoapySDRDevice_lastError()
ccall((:SoapySDRDevice_lastError, soapysdr), Ptr{Cchar}, ())
end
"""
SoapySDRKwargs
Definition for a key/value string map
"""
struct SoapySDRKwargs
size::Csize_t
keys::Ptr{Ptr{Cchar}}
vals::Ptr{Ptr{Cchar}}
end
"""
SoapySDRDevice_enumerate(args, length)
Enumerate a list of available devices on the system.
\\param args device construction key/value argument filters
\\param [out] length the number of elements in the result.
\\return a list of arguments strings, each unique to a device
"""
function SoapySDRDevice_enumerate(args, length)
@soapy_checked ccall(
(:SoapySDRDevice_enumerate, soapysdr),
Ptr{SoapySDRKwargs},
(Ptr{SoapySDRKwargs}, Ptr{Csize_t}),
args,
length,
)
end
"""
SoapySDRDevice_enumerateStrArgs(args, length)
Enumerate a list of available devices on the system.
Markup format for args: "keyA=valA, keyB=valB".
\\param args a markup string of key/value argument filters
\\param [out] length the number of elements in the result.
\\return a list of arguments strings, each unique to a device
"""
function SoapySDRDevice_enumerateStrArgs(args, length)
@soapy_checked ccall(
(:SoapySDRDevice_enumerateStrArgs, soapysdr),
Ptr{SoapySDRKwargs},
(Ptr{Cchar}, Ptr{Csize_t}),
args,
length,
)
end
"""
SoapySDRDevice_make(args)
Make a new Device object given device construction args.
The device pointer will be stored in a table so subsequent calls
with the same arguments will produce the same device.
For every call to make, there should be a matched call to unmake.
\\param args device construction key/value argument map
\\return a pointer to a new Device object
"""
function SoapySDRDevice_make(args)
@soapy_checked ccall(
(:SoapySDRDevice_make, soapysdr),
Ptr{SoapySDRDevice},
(Ptr{SoapySDRKwargs},),
args,
)
end
"""
SoapySDRDevice_makeStrArgs(args)
Make a new Device object given device construction args.
The device pointer will be stored in a table so subsequent calls
with the same arguments will produce the same device.
For every call to make, there should be a matched call to unmake.
\\param args a markup string of key/value arguments
\\return a pointer to a new Device object or null for error
"""
function SoapySDRDevice_makeStrArgs(args)
@soapy_checked ccall(
(:SoapySDRDevice_makeStrArgs, soapysdr),
Ptr{SoapySDRDevice},
(Ptr{Cchar},),
args,
)
end
"""
SoapySDRDevice_unmake(device)
Unmake or release a device object handle.
\\param device a pointer to a device object
\\return 0 for success or error code on failure
"""
function SoapySDRDevice_unmake(device)
@soapy_checked ccall(
(:SoapySDRDevice_unmake, soapysdr),
Cint,
(Ptr{SoapySDRDevice},),
device,
)
end
"""
SoapySDRDevice_make_list(argsList, length)
Create a list of devices from a list of construction arguments.
This is a convenience call to parallelize device construction,
and is fundamentally a parallel for loop of make(Kwargs).
\\param argsList a list of device arguments per each device
\\param length the length of the argsList array
\\return a list of device pointers per each specified argument
"""
function SoapySDRDevice_make_list(argsList, length)
@soapy_checked ccall(
(:SoapySDRDevice_make_list, soapysdr),
Ptr{Ptr{SoapySDRDevice}},
(Ptr{SoapySDRKwargs}, Csize_t),
argsList,
length,
)
end
"""
SoapySDRDevice_make_listStrArgs(argsList, length)
Create a list of devices from a list of construction arguments.
This is a convenience call to parallelize device construction,
and is fundamentally a parallel for loop of makeStrArgs(args).
\\param argsList a list of device arguments per each device
\\param length the length of the argsList array
\\return a list of device pointers per each specified argument
"""
function SoapySDRDevice_make_listStrArgs(argsList, length)
@soapy_checked ccall(
(:SoapySDRDevice_make_listStrArgs, soapysdr),
Ptr{Ptr{SoapySDRDevice}},
(Ptr{Ptr{Cchar}}, Csize_t),
argsList,
length,
)
end
"""
SoapySDRDevice_unmake_list(devices, length)
Unmake or release a list of device handles
and free the devices array memory as well.
This is a convenience call to parallelize device destruction,
and is fundamentally a parallel for loop of unmake(Device *).
\\param devices a list of pointers to device objects
\\param length the length of the devices array
\\return 0 for success or error code on failure
"""
function SoapySDRDevice_unmake_list(devices, length)
@soapy_checked ccall(
(:SoapySDRDevice_unmake_list, soapysdr),
Cint,
(Ptr{Ptr{SoapySDRDevice}}, Csize_t),
devices,
length,
)
end
"""
SoapySDRDevice_getDriverKey(device)
A key that uniquely identifies the device driver.
This key identifies the underlying implementation.
Several variants of a product may share a driver.
\\param device a pointer to a device instance
"""
function SoapySDRDevice_getDriverKey(device)
@soapy_checked ccall(
(:SoapySDRDevice_getDriverKey, soapysdr),
Ptr{Cchar},
(Ptr{SoapySDRDevice},),
device,
)
end
"""
SoapySDRDevice_getHardwareKey(device)
A key that uniquely identifies the hardware.
This key should be meaningful to the user
to optimize for the underlying hardware.
\\param device a pointer to a device instance
"""
function SoapySDRDevice_getHardwareKey(device)
if !isopen(device)
throw(InvalidStateException("Device is closed!", :closed))
end
@soapy_checked ccall(
(:SoapySDRDevice_getHardwareKey, soapysdr),
Ptr{Cchar},
(Ptr{SoapySDRDevice},),
device,
)
end
"""
SoapySDRDevice_getHardwareInfo(device)
Query a dictionary of available device information.
This dictionary can any number of values like
vendor name, product name, revisions, serials...
This information can be displayed to the user
to help identify the instantiated device.
\\param device a pointer to a device instance
"""
function SoapySDRDevice_getHardwareInfo(device)
@soapy_checked ccall(
(:SoapySDRDevice_getHardwareInfo, soapysdr),
SoapySDRKwargs,
(Ptr{SoapySDRDevice},),
device,
)
end
"""
SoapySDRDevice_setFrontendMapping(device, direction, mapping)
Set the frontend mapping of available DSP units to RF frontends.
This mapping controls channel mapping and channel availability.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param mapping a vendor-specific mapping string
\\return an error code or 0 for success
"""
function SoapySDRDevice_setFrontendMapping(device, direction, mapping)
@soapy_checked ccall(
(:SoapySDRDevice_setFrontendMapping, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Cint, Ptr{Cchar}),
device,
direction,
mapping,
)
end
"""
SoapySDRDevice_getFrontendMapping(device, direction)
Get the mapping configuration string.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\return the vendor-specific mapping string
"""
function SoapySDRDevice_getFrontendMapping(device, direction)
@soapy_checked ccall(
(:SoapySDRDevice_getFrontendMapping, soapysdr),
Ptr{Cchar},
(Ptr{SoapySDRDevice}, Cint),
device,
direction,
)
end
"""
SoapySDRDevice_getNumChannels(device, direction)
Get a number of channels given the streaming direction
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\return the number of channels
"""
function SoapySDRDevice_getNumChannels(device, direction)
@soapy_checked ccall(
(:SoapySDRDevice_getNumChannels, soapysdr),
Csize_t,
(Ptr{SoapySDRDevice}, Cint),
device,
direction,
)
end
"""
SoapySDRDevice_getChannelInfo(device, direction, channel)
Get channel info given the streaming direction
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel the channel number to get info for
\\return channel information
"""
function SoapySDRDevice_getChannelInfo(device, direction, channel)
@soapy_checked ccall(
(:SoapySDRDevice_getChannelInfo, soapysdr),
SoapySDRKwargs,
(Ptr{SoapySDRDevice}, Cint, Csize_t),
device,
direction,
channel,
)
end
"""
SoapySDRDevice_getFullDuplex(device, direction, channel)
Find out if the specified channel is full or half duplex.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\return true for full duplex, false for half duplex
"""
function SoapySDRDevice_getFullDuplex(device, direction, channel)
@soapy_checked ccall(
(:SoapySDRDevice_getFullDuplex, soapysdr),
Bool,
(Ptr{SoapySDRDevice}, Cint, Csize_t),
device,
direction,
channel,
)
end
"""
SoapySDRDevice_getStreamFormats(device, direction, channel, length)
Query a list of the available stream formats.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param [out] length the number of format strings
\\return a list of allowed format strings.
See SoapySDRDevice_setupStream() for the format syntax.
"""
function SoapySDRDevice_getStreamFormats(device, direction, channel, length)
@soapy_checked ccall(
(:SoapySDRDevice_getStreamFormats, soapysdr),
Ptr{Ptr{Cchar}},
(Ptr{SoapySDRDevice}, Cint, Csize_t, Ptr{Csize_t}),
device,
direction,
channel,
length,
)
end
"""
SoapySDRDevice_getNativeStreamFormat(device, direction, channel, fullScale)
Get the hardware's native stream format for this channel.
This is the format used by the underlying transport layer,
and the direct buffer access API calls (when available).
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param [out] fullScale the maximum possible value
\\return the native stream buffer format string
"""
function SoapySDRDevice_getNativeStreamFormat(device, direction, channel, fullScale)
@soapy_checked ccall(
(:SoapySDRDevice_getNativeStreamFormat, soapysdr),
Ptr{Cchar},
(Ptr{SoapySDRDevice}, Cint, Csize_t, Ptr{Cdouble}),
device,
direction,
channel,
fullScale,
)
end
"""
SoapySDRArgInfoType
Possible data types for argument info
"""
@cenum SoapySDRArgInfoType::UInt32 begin
SOAPY_SDR_ARG_INFO_BOOL = 0
SOAPY_SDR_ARG_INFO_INT = 1
SOAPY_SDR_ARG_INFO_FLOAT = 2
SOAPY_SDR_ARG_INFO_STRING = 3
end
"""
SoapySDRRange
Definition for a min/max numeric range
"""
struct SoapySDRRange
minimum::Cdouble
maximum::Cdouble
step::Cdouble
end
"""
SoapySDRArgInfo
Definition for argument info
"""
struct SoapySDRArgInfo
key::Ptr{Cchar}
value::Ptr{Cchar}
name::Ptr{Cchar}
description::Ptr{Cchar}
units::Ptr{Cchar}
type::SoapySDRArgInfoType
range::SoapySDRRange
numOptions::Csize_t
options::Ptr{Ptr{Cchar}}
optionNames::Ptr{Ptr{Cchar}}
end
"""
SoapySDRDevice_getStreamArgsInfo(device, direction, channel, length)
Query the argument info description for stream args.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param [out] length the number of argument infos
\\return a list of argument info structures
"""
function SoapySDRDevice_getStreamArgsInfo(device, direction, channel, length)
@soapy_checked ccall(
(:SoapySDRDevice_getStreamArgsInfo, soapysdr),
Ptr{SoapySDRArgInfo},
(Ptr{SoapySDRDevice}, Cint, Csize_t, Ptr{Csize_t}),
device,
direction,
channel,
length,
)
end
"""
SoapySDRDevice_setupStream(device, direction, format, channels, numChans, args)
Initialize a stream given a list of channels and stream arguments.
The implementation may change switches or power-up components.
All stream API calls should be usable with the new stream object
after setupStream() is complete, regardless of the activity state.
The API allows any number of simultaneous TX and RX streams, but many dual-channel
devices are limited to one stream in each direction, using either one or both channels.
This call will return an error if an unsupported combination is requested,
or if a requested channel in this direction is already in use by another stream.
When multiple channels are added to a stream, they are typically expected to have
the same sample rate. See SoapySDRDevice_setSampleRate().
\\param device a pointer to a device instance
\\return the opaque pointer to a stream handle.
\\parblock
The returned stream is not required to have internal locking, and may not be used
concurrently from multiple threads.
\\endparblock
\\param direction the channel direction (`SOAPY_SDR_RX` or `SOAPY_SDR_TX`)
\\param format A string representing the desired buffer format in read/writeStream()
\\parblock
The first character selects the number type:
- "C" means complex
- "F" means floating point
- "S" means signed integer
- "U" means unsigned integer
The type character is followed by the number of bits per number (complex is 2x this size per sample)
Example format strings:
- "CF32" - complex float32 (8 bytes per element)
- "CS16" - complex int16 (4 bytes per element)
- "CS12" - complex int12 (3 bytes per element)
- "CS4" - complex int4 (1 byte per element)
- "S32" - int32 (4 bytes per element)
- "U8" - uint8 (1 byte per element)
\\endparblock
\\param channels a list of channels or empty for automatic
\\param numChans the number of elements in the channels array
\\param args stream args or empty for defaults
\\parblock
Recommended keys to use in the args dictionary:
- "WIRE" - format of the samples between device and host
\\endparblock
\\return the stream pointer or nullptr for failure
"""
function SoapySDRDevice_setupStream(device, direction, format, channels, numChans, args)
@soapy_checked ccall(
(:SoapySDRDevice_setupStream, soapysdr),
Ptr{SoapySDRStream},
(Ptr{SoapySDRDevice}, Cint, Ptr{Cchar}, Ptr{Csize_t}, Csize_t, Ptr{SoapySDRKwargs}),
device,
direction,
format,
channels,
numChans,
args,
)
end
"""
SoapySDRDevice_closeStream(device, stream)
Close an open stream created by setupStream
\\param device a pointer to a device instance
\\param stream the opaque pointer to a stream handle
\\return 0 for success or error code on failure
"""
function SoapySDRDevice_closeStream(device, stream)
@soapy_checked ccall(
(:SoapySDRDevice_closeStream, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Ptr{SoapySDRStream}),
device,
stream,
)
end
"""
SoapySDRDevice_getStreamMTU(device, stream)
Get the stream's maximum transmission unit (MTU) in number of elements.
The MTU specifies the maximum payload transfer in a stream operation.
This value can be used as a stream buffer allocation size that can
best optimize throughput given the underlying stream implementation.
\\param device a pointer to a device instance
\\param stream the opaque pointer to a stream handle
\\return the MTU in number of stream elements (never zero)
"""
function SoapySDRDevice_getStreamMTU(device, stream)
@soapy_checked ccall(
(:SoapySDRDevice_getStreamMTU, soapysdr),
Csize_t,
(Ptr{SoapySDRDevice}, Ptr{SoapySDRStream}),
device,
stream,
)
end
"""
SoapySDRDevice_activateStream(device, stream, flags, timeNs, numElems)
Activate a stream.
Call activate to prepare a stream before using read/write().
The implementation control switches or stimulate data flow.
The timeNs is only valid when the flags have SOAPY_SDR_HAS_TIME.
The numElems count can be used to request a finite burst size.
The SOAPY_SDR_END_BURST flag can signal end on the finite burst.
Not all implementations will support the full range of options.
In this case, the implementation returns SOAPY_SDR_NOT_SUPPORTED.
\\param device a pointer to a device instance
\\param stream the opaque pointer to a stream handle
\\param flags optional flag indicators about the stream
\\param timeNs optional activation time in nanoseconds
\\param numElems optional element count for burst control
\\return 0 for success or error code on failure
"""
function SoapySDRDevice_activateStream(device, stream, flags, timeNs, numElems)
@soapy_checked ccall(
(:SoapySDRDevice_activateStream, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Ptr{SoapySDRStream}, Cint, Clonglong, Csize_t),
device,
stream,
flags,
timeNs,
numElems,
)
end
"""
SoapySDRDevice_deactivateStream(device, stream, flags, timeNs)
Deactivate a stream.
Call deactivate when not using using read/write().
The implementation control switches or halt data flow.
The timeNs is only valid when the flags have SOAPY_SDR_HAS_TIME.
Not all implementations will support the full range of options.
In this case, the implementation returns SOAPY_SDR_NOT_SUPPORTED.
\\param device a pointer to a device instance
\\param stream the opaque pointer to a stream handle
\\param flags optional flag indicators about the stream
\\param timeNs optional deactivation time in nanoseconds
\\return 0 for success or error code on failure
"""
function SoapySDRDevice_deactivateStream(device, stream, flags, timeNs)
if !isopen(stream)
throw(InvalidStateException("Stream is closed!", :closed))
end
@soapy_checked ccall(
(:SoapySDRDevice_deactivateStream, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Ptr{SoapySDRStream}, Cint, Clonglong),
device,
stream,
flags,
timeNs,
)
end
"""
SoapySDRDevice_readStream(device, stream, buffs, numElems, flags, timeNs, timeoutUs)
Read elements from a stream for reception.
This is a multi-channel call, and buffs should be an array of void *,
where each pointer will be filled with data from a different channel.
**Client code compatibility:**
The readStream() call should be well defined at all times,
including prior to activation and after deactivation.
When inactive, readStream() should implement the timeout
specified by the caller and return SOAPY_SDR_TIMEOUT.
\\param device a pointer to a device instance
\\param stream the opaque pointer to a stream handle
\\param buffs an array of void* buffers num chans in size
\\param numElems the number of elements in each buffer
\\param [out] flags optional flag indicators about the result
\\param [out] timeNs the buffer's timestamp in nanoseconds
\\param timeoutUs the timeout in microseconds
\\return the number of elements read per buffer or error code
"""
function SoapySDRDevice_readStream(
device,
stream,
buffs,
numElems,
flags,
timeNs,
timeoutUs,
)
@soapy_checked ccall(
(:SoapySDRDevice_readStream, soapysdr),
Cint,
(
Ptr{SoapySDRDevice},
Ptr{SoapySDRStream},
Ptr{Ptr{Cvoid}},
Csize_t,
Ptr{Cint},
Ptr{Clonglong},
Clong,
),
device,
stream,
buffs,
numElems,
flags,
timeNs,
timeoutUs,
)
end
"""
SoapySDRDevice_writeStream(device, stream, buffs, numElems, flags, timeNs, timeoutUs)
Write elements to a stream for transmission.
This is a multi-channel call, and buffs should be an array of void *,
where each pointer will be filled with data for a different channel.
**Client code compatibility:**
Client code relies on writeStream() for proper back-pressure.
The writeStream() implementation must enforce the timeout
such that the call blocks until space becomes available
or timeout expiration.
\\param device a pointer to a device instance
\\param stream the opaque pointer to a stream handle
\\param buffs an array of void* buffers num chans in size
\\param numElems the number of elements in each buffer
\\param [in,out] flags optional input flags and output flags
\\param timeNs the buffer's timestamp in nanoseconds
\\param timeoutUs the timeout in microseconds
\\return the number of elements written per buffer or error
"""
function SoapySDRDevice_writeStream(
device,
stream,
buffs,
numElems,
flags,
timeNs,
timeoutUs,
)
@soapy_checked ccall(
(:SoapySDRDevice_writeStream, soapysdr),
Cint,
(
Ptr{SoapySDRDevice},
Ptr{SoapySDRStream},
Ptr{Ptr{Cvoid}},
Csize_t,
Ptr{Cint},
Clonglong,
Clong,
),
device,
stream,
buffs,
numElems,
flags,
timeNs,
timeoutUs,
)
end
"""
SoapySDRDevice_readStreamStatus(device, stream, chanMask, flags, timeNs, timeoutUs)
Readback status information about a stream.
This call is typically used on a transmit stream
to report time errors, underflows, and burst completion.
**Client code compatibility:**
Client code may continually poll readStreamStatus() in a loop.
Implementations of readStreamStatus() should wait in the call
for a status change event or until the timeout expiration.
When stream status is not implemented on a particular stream,
readStreamStatus() should return SOAPY_SDR_NOT_SUPPORTED.
Client code may use this indication to disable a polling loop.
\\param device a pointer to a device instance
\\param stream the opaque pointer to a stream handle
\\param chanMask to which channels this status applies
\\param flags optional input flags and output flags
\\param timeNs the buffer's timestamp in nanoseconds
\\param timeoutUs the timeout in microseconds
\\return 0 for success or error code like timeout
"""
function SoapySDRDevice_readStreamStatus(device, stream, chanMask, flags, timeNs, timeoutUs)
@soapy_checked ccall(
(:SoapySDRDevice_readStreamStatus, soapysdr),
Cint,
(
Ptr{SoapySDRDevice},
Ptr{SoapySDRStream},
Ptr{Csize_t},
Ptr{Cint},
Ptr{Clonglong},
Clong,
),
device,
stream,
chanMask,
flags,
timeNs,
timeoutUs,
)
end
"""
SoapySDRDevice_getNumDirectAccessBuffers(device, stream)
How many direct access buffers can the stream provide?
This is the number of times the user can call acquire()
on a stream without making subsequent calls to release().
A return value of 0 means that direct access is not supported.
\\param device a pointer to a device instance
\\param stream the opaque pointer to a stream handle
\\return the number of direct access buffers or 0
"""
function SoapySDRDevice_getNumDirectAccessBuffers(device, stream)
@soapy_checked ccall(
(:SoapySDRDevice_getNumDirectAccessBuffers, soapysdr),
Csize_t,
(Ptr{SoapySDRDevice}, Ptr{SoapySDRStream}),
device,
stream,
)
end
"""
SoapySDRDevice_getDirectAccessBufferAddrs(device, stream, handle, buffs)
Get the buffer addresses for a scatter/gather table entry.
When the underlying DMA implementation uses scatter/gather
then this call provides the user addresses for that table.
Example: The caller may query the DMA memory addresses once
after stream creation to pre-allocate a re-usable ring-buffer.
\\param device a pointer to a device instance
\\param stream the opaque pointer to a stream handle
\\param handle an index value between 0 and num direct buffers - 1
\\param buffs an array of void* buffers num chans in size
\\return 0 for success or error code when not supported
"""
function SoapySDRDevice_getDirectAccessBufferAddrs(device, stream, handle, buffs)
@soapy_checked ccall(
(:SoapySDRDevice_getDirectAccessBufferAddrs, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Ptr{SoapySDRStream}, Csize_t, Ptr{Ptr{Cvoid}}),
device,
stream,
handle,
buffs,
)
end
"""
SoapySDRDevice_acquireReadBuffer(device, stream, handle, buffs, flags, timeNs, timeoutUs)
Acquire direct buffers from a receive stream.
This call is part of the direct buffer access API.
The buffs array will be filled with a stream pointer for each channel.
Each pointer can be read up to the number of return value elements.
The handle will be set by the implementation so that the caller
may later release access to the buffers with releaseReadBuffer().
Handle represents an index into the internal scatter/gather table
such that handle is between 0 and num direct buffers - 1.
\\param device a pointer to a device instance
\\param stream the opaque pointer to a stream handle
\\param handle an index value used in the release() call
\\param buffs an array of void* buffers num chans in size
\\param flags optional flag indicators about the result
\\param timeNs the buffer's timestamp in nanoseconds
\\param timeoutUs the timeout in microseconds
\\return the number of elements read per buffer or error code
"""
function SoapySDRDevice_acquireReadBuffer(
device,
stream,
handle,
buffs,
flags,
timeNs,
timeoutUs,
)
@soapy_checked ccall(
(:SoapySDRDevice_acquireReadBuffer, soapysdr),
Cint,
(
Ptr{SoapySDRDevice},
Ptr{SoapySDRStream},
Ptr{Csize_t},
Ptr{Ptr{Cvoid}},
Ptr{Cint},
Ptr{Clonglong},
Clong,
),
device,
stream,
handle,
buffs,
flags,
timeNs,
timeoutUs,
)
end
"""
SoapySDRDevice_releaseReadBuffer(device, stream, handle)
Release an acquired buffer back to the receive stream.
This call is part of the direct buffer access API.
\\param device a pointer to a device instance
\\param stream the opaque pointer to a stream handle
\\param handle the opaque handle from the acquire() call
"""
function SoapySDRDevice_releaseReadBuffer(device, stream, handle)
@soapy_checked ccall(
(:SoapySDRDevice_releaseReadBuffer, soapysdr),
Cvoid,
(Ptr{SoapySDRDevice}, Ptr{SoapySDRStream}, Csize_t),
device,
stream,
handle,
)
end
"""
SoapySDRDevice_acquireWriteBuffer(device, stream, handle, buffs, timeoutUs)
Acquire direct buffers from a transmit stream.
This call is part of the direct buffer access API.
The buffs array will be filled with a stream pointer for each channel.
Each pointer can be written up to the number of return value elements.
The handle will be set by the implementation so that the caller
may later release access to the buffers with releaseWriteBuffer().
Handle represents an index into the internal scatter/gather table
such that handle is between 0 and num direct buffers - 1.
\\param device a pointer to a device instance
\\param stream the opaque pointer to a stream handle
\\param handle an index value used in the release() call
\\param buffs an array of void* buffers num chans in size
\\param timeoutUs the timeout in microseconds
\\return the number of available elements per buffer or error
"""
function SoapySDRDevice_acquireWriteBuffer(device, stream, handle, buffs, timeoutUs)
@soapy_checked ccall(
(:SoapySDRDevice_acquireWriteBuffer, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Ptr{SoapySDRStream}, Ptr{Csize_t}, Ptr{Ptr{Cvoid}}, Clong),
device,
stream,
handle,
buffs,
timeoutUs,
)
end
"""
SoapySDRDevice_releaseWriteBuffer(device, stream, handle, numElems, flags, timeNs)
Release an acquired buffer back to the transmit stream.
This call is part of the direct buffer access API.
Stream meta-data is provided as part of the release call,
and not the acquire call so that the caller may acquire
buffers without committing to the contents of the meta-data,
which can be determined by the user as the buffers are filled.
\\param device a pointer to a device instance
\\param stream the opaque pointer to a stream handle
\\param handle the opaque handle from the acquire() call
\\param numElems the number of elements written to each buffer
\\param flags optional input flags and output flags
\\param timeNs the buffer's timestamp in nanoseconds
"""
function SoapySDRDevice_releaseWriteBuffer(device, stream, handle, numElems, flags, timeNs)
@soapy_checked ccall(
(:SoapySDRDevice_releaseWriteBuffer, soapysdr),
Cvoid,
(Ptr{SoapySDRDevice}, Ptr{SoapySDRStream}, Csize_t, Csize_t, Ptr{Cint}, Clonglong),
device,
stream,
handle,
numElems,
flags,
timeNs,
)
end
"""
SoapySDRDevice_listAntennas(device, direction, channel, length)
Get a list of available antennas to select on a given chain.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param [out] length the number of antenna names
\\return a list of available antenna names
"""
function SoapySDRDevice_listAntennas(device, direction, channel, length)
@soapy_checked ccall(
(:SoapySDRDevice_listAntennas, soapysdr),
Ptr{Ptr{Cchar}},
(Ptr{SoapySDRDevice}, Cint, Csize_t, Ptr{Csize_t}),
device,
direction,
channel,
length,
)
end
"""
SoapySDRDevice_setAntenna(device, direction, channel, name)
Set the selected antenna on a chain.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param name the name of an available antenna
\\return an error code or 0 for success
"""
function SoapySDRDevice_setAntenna(device, direction, channel, name)
@soapy_checked ccall(
(:SoapySDRDevice_setAntenna, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Cint, Csize_t, Ptr{Cchar}),
device,
direction,
channel,
name,
)
end
"""
SoapySDRDevice_getAntenna(device, direction, channel)
Get the selected antenna on a chain.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\return the name of an available antenna
"""
function SoapySDRDevice_getAntenna(device, direction, channel)
@soapy_checked ccall(
(:SoapySDRDevice_getAntenna, soapysdr),
Ptr{Cchar},
(Ptr{SoapySDRDevice}, Cint, Csize_t),
device,
direction,
channel,
)
end
"""
SoapySDRDevice_hasDCOffsetMode(device, direction, channel)
Does the device support automatic DC offset corrections?
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\return true if automatic corrections are supported
"""
function SoapySDRDevice_hasDCOffsetMode(device, direction, channel)
@soapy_checked ccall(
(:SoapySDRDevice_hasDCOffsetMode, soapysdr),
Bool,
(Ptr{SoapySDRDevice}, Cint, Csize_t),
device,
direction,
channel,
)
end
"""
SoapySDRDevice_setDCOffsetMode(device, direction, channel, automatic)
Set the automatic DC offset corrections mode.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param automatic true for automatic offset correction
\\return an error code or 0 for success
"""
function SoapySDRDevice_setDCOffsetMode(device, direction, channel, automatic)
@soapy_checked ccall(
(:SoapySDRDevice_setDCOffsetMode, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Cint, Csize_t, Bool),
device,
direction,
channel,
automatic,
)
end
"""
SoapySDRDevice_getDCOffsetMode(device, direction, channel)
Get the automatic DC offset corrections mode.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\return true for automatic offset correction
"""
function SoapySDRDevice_getDCOffsetMode(device, direction, channel)
@soapy_checked ccall(
(:SoapySDRDevice_getDCOffsetMode, soapysdr),
Bool,
(Ptr{SoapySDRDevice}, Cint, Csize_t),
device,
direction,
channel,
)
end
"""
SoapySDRDevice_hasDCOffset(device, direction, channel)
Does the device support frontend DC offset correction?
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\return true if DC offset corrections are supported
"""
function SoapySDRDevice_hasDCOffset(device, direction, channel)
@soapy_checked ccall(
(:SoapySDRDevice_hasDCOffset, soapysdr),
Bool,
(Ptr{SoapySDRDevice}, Cint, Csize_t),
device,
direction,
channel,
)
end
"""
SoapySDRDevice_setDCOffset(device, direction, channel, offsetI, offsetQ)
Set the frontend DC offset correction.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param offsetI the relative correction (1.0 max)
\\param offsetQ the relative correction (1.0 max)
\\return an error code or 0 for success
"""
function SoapySDRDevice_setDCOffset(device, direction, channel, offsetI, offsetQ)
@soapy_checked ccall(
(:SoapySDRDevice_setDCOffset, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Cint, Csize_t, Cdouble, Cdouble),
device,
direction,
channel,
offsetI,
offsetQ,
)
end
"""
SoapySDRDevice_getDCOffset(device, direction, channel, offsetI, offsetQ)
Get the frontend DC offset correction.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param [out] offsetI the relative correction (1.0 max)
\\param [out] offsetQ the relative correction (1.0 max)
\\return 0 for success or error code on failure
"""
function SoapySDRDevice_getDCOffset(device, direction, channel, offsetI, offsetQ)
@soapy_checked ccall(
(:SoapySDRDevice_getDCOffset, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Cint, Csize_t, Ptr{Cdouble}, Ptr{Cdouble}),
device,
direction,
channel,
offsetI,
offsetQ,
)
end
"""
SoapySDRDevice_hasIQBalance(device, direction, channel)
Does the device support frontend IQ balance correction?
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\return true if IQ balance corrections are supported
"""
function SoapySDRDevice_hasIQBalance(device, direction, channel)
@soapy_checked ccall(
(:SoapySDRDevice_hasIQBalance, soapysdr),
Bool,
(Ptr{SoapySDRDevice}, Cint, Csize_t),
device,
direction,
channel,
)
end
"""
SoapySDRDevice_setIQBalance(device, direction, channel, balanceI, balanceQ)
Set the frontend IQ balance correction.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param balanceI the relative correction (1.0 max)
\\param balanceQ the relative correction (1.0 max)
\\return an error code or 0 for success
"""
function SoapySDRDevice_setIQBalance(device, direction, channel, balanceI, balanceQ)
@soapy_checked ccall(
(:SoapySDRDevice_setIQBalance, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Cint, Csize_t, Cdouble, Cdouble),
device,
direction,
channel,
balanceI,
balanceQ,
)
end
"""
SoapySDRDevice_getIQBalance(device, direction, channel, balanceI, balanceQ)
Get the frontend IQ balance correction.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param [out] balanceI the relative correction (1.0 max)
\\param [out] balanceQ the relative correction (1.0 max)
\\return 0 for success or error code on failure
"""
function SoapySDRDevice_getIQBalance(device, direction, channel, balanceI, balanceQ)
@soapy_checked ccall(
(:SoapySDRDevice_getIQBalance, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Cint, Csize_t, Ptr{Cdouble}, Ptr{Cdouble}),
device,
direction,
channel,
balanceI,
balanceQ,
)
end
"""
SoapySDRDevice_hasIQBalanceMode(device, direction, channel)
Does the device support automatic frontend IQ balance correction?
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\return true if automatic IQ balance corrections are supported
"""
function SoapySDRDevice_hasIQBalanceMode(device, direction, channel)
@soapy_checked ccall(
(:SoapySDRDevice_hasIQBalanceMode, soapysdr),
Bool,
(Ptr{SoapySDRDevice}, Cint, Csize_t),
device,
direction,
channel,
)
end
"""
SoapySDRDevice_setIQBalanceMode(device, direction, channel, automatic)
Set the automatic frontend IQ balance correction.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param automatic true for automatic correction
\\return 0 for success or error code on failure
"""
function SoapySDRDevice_setIQBalanceMode(device, direction, channel, automatic)
@soapy_checked ccall(
(:SoapySDRDevice_setIQBalanceMode, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Cint, Csize_t, Bool),
device,
direction,
channel,
automatic,
)
end
"""
SoapySDRDevice_getIQBalanceMode(device, direction, channel)
Get the automatic frontend IQ balance corrections mode.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\return true for automatic correction
"""
function SoapySDRDevice_getIQBalanceMode(device, direction, channel)
@soapy_checked ccall(
(:SoapySDRDevice_getIQBalanceMode, soapysdr),
Bool,
(Ptr{SoapySDRDevice}, Cint, Csize_t),
device,
direction,
channel,
)
end
"""
SoapySDRDevice_hasFrequencyCorrection(device, direction, channel)
Does the device support frontend frequency correction?
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\return true if frequency corrections are supported
"""
function SoapySDRDevice_hasFrequencyCorrection(device, direction, channel)
@soapy_checked ccall(
(:SoapySDRDevice_hasFrequencyCorrection, soapysdr),
Bool,
(Ptr{SoapySDRDevice}, Cint, Csize_t),
device,
direction,
channel,
)
end
"""
SoapySDRDevice_setFrequencyCorrection(device, direction, channel, value)
Fine tune the frontend frequency correction.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param value the correction in PPM
\\return an error code or 0 for success
"""
function SoapySDRDevice_setFrequencyCorrection(device, direction, channel, value)
@soapy_checked ccall(
(:SoapySDRDevice_setFrequencyCorrection, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Cint, Csize_t, Cdouble),
device,
direction,
channel,
value,
)
end
"""
SoapySDRDevice_getFrequencyCorrection(device, direction, channel)
Get the frontend frequency correction value.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\return the correction value in PPM
"""
function SoapySDRDevice_getFrequencyCorrection(device, direction, channel)
@soapy_checked ccall(
(:SoapySDRDevice_getFrequencyCorrection, soapysdr),
Cdouble,
(Ptr{SoapySDRDevice}, Cint, Csize_t),
device,
direction,
channel,
)
end
"""
SoapySDRDevice_listGains(device, direction, channel, length)
List available amplification elements.
Elements should be in order RF to baseband.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel
\\param [out] length the number of gain names
\\return a list of gain string names
"""
function SoapySDRDevice_listGains(device, direction, channel, length)
@soapy_checked ccall(
(:SoapySDRDevice_listGains, soapysdr),
Ptr{Ptr{Cchar}},
(Ptr{SoapySDRDevice}, Cint, Csize_t, Ptr{Csize_t}),
device,
direction,
channel,
length,
)
end
"""
SoapySDRDevice_hasGainMode(device, direction, channel)
Does the device support automatic gain control?
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\return true for automatic gain control
"""
function SoapySDRDevice_hasGainMode(device, direction, channel)
@soapy_checked ccall(
(:SoapySDRDevice_hasGainMode, soapysdr),
Bool,
(Ptr{SoapySDRDevice}, Cint, Csize_t),
device,
direction,
channel,
)
end
"""
SoapySDRDevice_setGainMode(device, direction, channel, automatic)
Set the automatic gain mode on the chain.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param automatic true for automatic gain setting
\\return an error code or 0 for success
"""
function SoapySDRDevice_setGainMode(device, direction, channel, automatic)
@soapy_checked ccall(
(:SoapySDRDevice_setGainMode, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Cint, Csize_t, Bool),
device,
direction,
channel,
automatic,
)
end
"""
SoapySDRDevice_getGainMode(device, direction, channel)
Get the automatic gain mode on the chain.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\return true for automatic gain setting
"""
function SoapySDRDevice_getGainMode(device, direction, channel)
@soapy_checked ccall(
(:SoapySDRDevice_getGainMode, soapysdr),
Bool,
(Ptr{SoapySDRDevice}, Cint, Csize_t),
device,
direction,
channel,
)
end
"""
SoapySDRDevice_setGain(device, direction, channel, value)
Set the overall amplification in a chain.
The gain will be distributed automatically across available element.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param value the new amplification value in dB
\\return an error code or 0 for success
"""
function SoapySDRDevice_setGain(device, direction, channel, value)
@soapy_checked ccall(
(:SoapySDRDevice_setGain, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Cint, Csize_t, Cdouble),
device,
direction,
channel,
value,
)
end
"""
SoapySDRDevice_setGainElement(device, direction, channel, name, value)
Set the value of a amplification element in a chain.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param name the name of an amplification element
\\param value the new amplification value in dB
\\return an error code or 0 for success
"""
function SoapySDRDevice_setGainElement(device, direction, channel, name, value)
@soapy_checked ccall(
(:SoapySDRDevice_setGainElement, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Cint, Csize_t, Ptr{Cchar}, Cdouble),
device,
direction,
channel,
name,
value,
)
end
"""
SoapySDRDevice_getGain(device, direction, channel)
Get the overall value of the gain elements in a chain.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\return the value of the gain in dB
"""
function SoapySDRDevice_getGain(device, direction, channel)
@soapy_checked ccall(
(:SoapySDRDevice_getGain, soapysdr),
Cdouble,
(Ptr{SoapySDRDevice}, Cint, Csize_t),
device,
direction,
channel,
)
end
"""
SoapySDRDevice_getGainElement(device, direction, channel, name)
Get the value of an individual amplification element in a chain.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param name the name of an amplification element
\\return the value of the gain in dB
"""
function SoapySDRDevice_getGainElement(device, direction, channel, name)
@soapy_checked ccall(
(:SoapySDRDevice_getGainElement, soapysdr),
Cdouble,
(Ptr{SoapySDRDevice}, Cint, Csize_t, Ptr{Cchar}),
device,
direction,
channel,
name,
)
end
"""
SoapySDRDevice_getGainRange(device, direction, channel)
Get the overall range of possible gain values.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\return the range of possible gain values for this channel in dB
"""
function SoapySDRDevice_getGainRange(device, direction, channel)
@soapy_checked ccall(
(:SoapySDRDevice_getGainRange, soapysdr),
SoapySDRRange,
(Ptr{SoapySDRDevice}, Cint, Csize_t),
device,
direction,
channel,
)
end
"""
SoapySDRDevice_getGainElementRange(device, direction, channel, name)
Get the range of possible gain values for a specific element.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param name the name of an amplification element
\\return the range of possible gain values for the specified amplification element in dB
"""
function SoapySDRDevice_getGainElementRange(device, direction, channel, name)
@soapy_checked ccall(
(:SoapySDRDevice_getGainElementRange, soapysdr),
SoapySDRRange,
(Ptr{SoapySDRDevice}, Cint, Csize_t, Ptr{Cchar}),
device,
direction,
channel,
name,
)
end
"""
SoapySDRDevice_setFrequency(device, direction, channel, frequency, args)
Set the center frequency of the chain.
- For RX, this specifies the down-conversion frequency.
- For TX, this specifies the up-conversion frequency.
The default implementation of setFrequency() will tune the "RF"
component as close as possible to the requested center frequency.
Tuning inaccuracies will be compensated for with the "BB" component.
The args can be used to augment the tuning algorithm.
- Use "OFFSET" to specify an "RF" tuning offset,
usually with the intention of moving the LO out of the passband.
The offset will be compensated for using the "BB" component.
- Use the name of a component for the key and a frequency in Hz
as the value (any format) to enforce a specific frequency.
The other components will be tuned with compensation
to achieve the specified overall frequency.
- Use the name of a component for the key and the value "IGNORE"
so that the tuning algorithm will avoid altering the component.
- Vendor specific implementations can also use the same args to augment
tuning in other ways such as specifying fractional vs integer N tuning.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param frequency the center frequency in Hz
\\param args optional tuner arguments
\\return an error code or 0 for success
"""
function SoapySDRDevice_setFrequency(device, direction, channel, frequency, args)
@soapy_checked ccall(
(:SoapySDRDevice_setFrequency, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Cint, Csize_t, Cdouble, Ptr{SoapySDRKwargs}),
device,
direction,
channel,
frequency,
args,
)
end
"""
SoapySDRDevice_setFrequencyComponent(device, direction, channel, name, frequency, args)
Tune the center frequency of the specified element.
- For RX, this specifies the down-conversion frequency.
- For TX, this specifies the up-conversion frequency.
Recommended names used to represent tunable components:
- "CORR" - freq error correction in PPM
- "RF" - frequency of the RF frontend
- "BB" - frequency of the baseband DSP
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param name the name of a tunable element
\\param frequency the center frequency in Hz
\\param args optional tuner arguments
\\return an error code or 0 for success
"""
function SoapySDRDevice_setFrequencyComponent(
device,
direction,
channel,
name,
frequency,
args,
)
@soapy_checked ccall(
(:SoapySDRDevice_setFrequencyComponent, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Cint, Csize_t, Ptr{Cchar}, Cdouble, Ptr{SoapySDRKwargs}),
device,
direction,
channel,
name,
frequency,
args,
)
end
"""
SoapySDRDevice_getFrequency(device, direction, channel)
Get the overall center frequency of the chain.
- For RX, this specifies the down-conversion frequency.
- For TX, this specifies the up-conversion frequency.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\return the center frequency in Hz
"""
function SoapySDRDevice_getFrequency(device, direction, channel)
@soapy_checked ccall(
(:SoapySDRDevice_getFrequency, soapysdr),
Cdouble,
(Ptr{SoapySDRDevice}, Cint, Csize_t),
device,
direction,
channel,
)
end
"""
SoapySDRDevice_getFrequencyComponent(device, direction, channel, name)
Get the frequency of a tunable element in the chain.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param name the name of a tunable element
\\return the tunable element's frequency in Hz
"""
function SoapySDRDevice_getFrequencyComponent(device, direction, channel, name)
@soapy_checked ccall(
(:SoapySDRDevice_getFrequencyComponent, soapysdr),
Cdouble,
(Ptr{SoapySDRDevice}, Cint, Csize_t, Ptr{Cchar}),
device,
direction,
channel,
name,
)
end
"""
SoapySDRDevice_listFrequencies(device, direction, channel, length)
List available tunable elements in the chain.
Elements should be in order RF to baseband.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel
\\param [out] length the number names
\\return a list of tunable elements by name
"""
function SoapySDRDevice_listFrequencies(device, direction, channel, length)
@soapy_checked ccall(
(:SoapySDRDevice_listFrequencies, soapysdr),
Ptr{Ptr{Cchar}},
(Ptr{SoapySDRDevice}, Cint, Csize_t, Ptr{Csize_t}),
device,
direction,
channel,
length,
)
end
"""
SoapySDRDevice_getFrequencyRange(device, direction, channel, length)
Get the range of overall frequency values.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param [out] length the number of ranges
\\return a list of frequency ranges in Hz
"""
function SoapySDRDevice_getFrequencyRange(device, direction, channel, length)
@soapy_checked ccall(
(:SoapySDRDevice_getFrequencyRange, soapysdr),
Ptr{SoapySDRRange},
(Ptr{SoapySDRDevice}, Cint, Csize_t, Ptr{Csize_t}),
device,
direction,
channel,
length,
)
end
"""
SoapySDRDevice_getFrequencyRangeComponent(device, direction, channel, name, length)
Get the range of tunable values for the specified element.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param name the name of a tunable element
\\param [out] length the number of ranges
\\return a list of frequency ranges in Hz
"""
function SoapySDRDevice_getFrequencyRangeComponent(device, direction, channel, name, length)
@soapy_checked ccall(
(:SoapySDRDevice_getFrequencyRangeComponent, soapysdr),
Ptr{SoapySDRRange},
(Ptr{SoapySDRDevice}, Cint, Csize_t, Ptr{Cchar}, Ptr{Csize_t}),
device,
direction,
channel,
name,
length,
)
end
"""
SoapySDRDevice_getFrequencyArgsInfo(device, direction, channel, length)
Query the argument info description for tune args.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param [out] length the number of argument infos
\\return a list of argument info structures
"""
function SoapySDRDevice_getFrequencyArgsInfo(device, direction, channel, length)
@soapy_checked ccall(
(:SoapySDRDevice_getFrequencyArgsInfo, soapysdr),
Ptr{SoapySDRArgInfo},
(Ptr{SoapySDRDevice}, Cint, Csize_t, Ptr{Csize_t}),
device,
direction,
channel,
length,
)
end
"""
SoapySDRDevice_setSampleRate(device, direction, channel, rate)
Set the baseband sample rate of the chain.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param rate the sample rate in samples per second
\\return an error code or 0 for success
"""
function SoapySDRDevice_setSampleRate(device, direction, channel, rate)
@soapy_checked ccall(
(:SoapySDRDevice_setSampleRate, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Cint, Csize_t, Cdouble),
device,
direction,
channel,
rate,
)
end
"""
SoapySDRDevice_getSampleRate(device, direction, channel)
Get the baseband sample rate of the chain.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\return the sample rate in samples per second
"""
function SoapySDRDevice_getSampleRate(device, direction, channel)
@soapy_checked ccall(
(:SoapySDRDevice_getSampleRate, soapysdr),
Cdouble,
(Ptr{SoapySDRDevice}, Cint, Csize_t),
device,
direction,
channel,
)
end
"""
SoapySDRDevice_listSampleRates(device, direction, channel, length)
Get the range of possible baseband sample rates.
\\deprecated replaced by getSampleRateRange()
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param [out] length the number of sample rates
\\return a list of possible rates in samples per second
"""
function SoapySDRDevice_listSampleRates(device, direction, channel, length)
@soapy_checked ccall(
(:SoapySDRDevice_listSampleRates, soapysdr),
Ptr{Cdouble},
(Ptr{SoapySDRDevice}, Cint, Csize_t, Ptr{Csize_t}),
device,
direction,
channel,
length,
)
end
"""
SoapySDRDevice_getSampleRateRange(device, direction, channel, length)
Get the range of possible baseband sample rates.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param [out] length the number of sample rates
\\return a list of sample rate ranges in samples per second
"""
function SoapySDRDevice_getSampleRateRange(device, direction, channel, length)
@soapy_checked ccall(
(:SoapySDRDevice_getSampleRateRange, soapysdr),
Ptr{SoapySDRRange},
(Ptr{SoapySDRDevice}, Cint, Csize_t, Ptr{Csize_t}),
device,
direction,
channel,
length,
)
end
"""
SoapySDRDevice_setBandwidth(device, direction, channel, bw)
Set the baseband filter width of the chain.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param bw the baseband filter width in Hz
\\return an error code or 0 for success
"""
function SoapySDRDevice_setBandwidth(device, direction, channel, bw)
@soapy_checked ccall(
(:SoapySDRDevice_setBandwidth, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Cint, Csize_t, Cdouble),
device,
direction,
channel,
bw,
)
end
"""
SoapySDRDevice_getBandwidth(device, direction, channel)
Get the baseband filter width of the chain.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\return the baseband filter width in Hz
"""
function SoapySDRDevice_getBandwidth(device, direction, channel)
@soapy_checked ccall(
(:SoapySDRDevice_getBandwidth, soapysdr),
Cdouble,
(Ptr{SoapySDRDevice}, Cint, Csize_t),
device,
direction,
channel,
)
end
"""
SoapySDRDevice_listBandwidths(device, direction, channel, length)
Get the range of possible baseband filter widths.
\\deprecated replaced by getBandwidthRange()
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param [out] length the number of bandwidths
\\return a list of possible bandwidths in Hz
"""
function SoapySDRDevice_listBandwidths(device, direction, channel, length)
@soapy_checked ccall(
(:SoapySDRDevice_listBandwidths, soapysdr),
Ptr{Cdouble},
(Ptr{SoapySDRDevice}, Cint, Csize_t, Ptr{Csize_t}),
device,
direction,
channel,
length,
)
end
"""
SoapySDRDevice_getBandwidthRange(device, direction, channel, length)
Get the range of possible baseband filter widths.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param [out] length the number of ranges
\\return a list of bandwidth ranges in Hz
"""
function SoapySDRDevice_getBandwidthRange(device, direction, channel, length)
@soapy_checked ccall(
(:SoapySDRDevice_getBandwidthRange, soapysdr),
Ptr{SoapySDRRange},
(Ptr{SoapySDRDevice}, Cint, Csize_t, Ptr{Csize_t}),
device,
direction,
channel,
length,
)
end
"""
SoapySDRDevice_setMasterClockRate(device, rate)
Set the master clock rate of the device.
\\param device a pointer to a device instance
\\param rate the clock rate in Hz
\\return an error code or 0 for success
"""
function SoapySDRDevice_setMasterClockRate(device, rate)
@soapy_checked ccall(
(:SoapySDRDevice_setMasterClockRate, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Cdouble),
device,
rate,
)
end
"""
SoapySDRDevice_getMasterClockRate(device)
Get the master clock rate of the device.
\\param device a pointer to a device instance
\\return the clock rate in Hz
"""
function SoapySDRDevice_getMasterClockRate(device)
@soapy_checked ccall(
(:SoapySDRDevice_getMasterClockRate, soapysdr),
Cdouble,
(Ptr{SoapySDRDevice},),
device,
)
end
"""
SoapySDRDevice_getMasterClockRates(device, length)
Get the range of available master clock rates.
\\param device a pointer to a device instance
\\param [out] length the number of ranges
\\return a list of clock rate ranges in Hz
"""
function SoapySDRDevice_getMasterClockRates(device, length)
@soapy_checked ccall(
(:SoapySDRDevice_getMasterClockRates, soapysdr),
Ptr{SoapySDRRange},
(Ptr{SoapySDRDevice}, Ptr{Csize_t}),
device,
length,
)
end
"""
SoapySDRDevice_setReferenceClockRate(device, rate)
Set the reference clock rate of the device.
\\param device a pointer to a device instance
\\param rate the clock rate in Hz
\\return an error code or 0 for success
"""
function SoapySDRDevice_setReferenceClockRate(device, rate)
@soapy_checked ccall(
(:SoapySDRDevice_setReferenceClockRate, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Cdouble),
device,
rate,
)
end
"""
SoapySDRDevice_getReferenceClockRate(device)
Get the reference clock rate of the device.
\\param device a pointer to a device instance
\\return the clock rate in Hz
"""
function SoapySDRDevice_getReferenceClockRate(device)
@soapy_checked ccall(
(:SoapySDRDevice_getReferenceClockRate, soapysdr),
Cdouble,
(Ptr{SoapySDRDevice},),
device,
)
end
"""
SoapySDRDevice_getReferenceClockRates(device, length)
Get the range of available reference clock rates.
\\param device a pointer to a device instance
\\param [out] length the number of sources
\\return a list of clock rate ranges in Hz
"""
function SoapySDRDevice_getReferenceClockRates(device, length)
ccall(
(:SoapySDRDevice_getReferenceClockRates, soapysdr),
Ptr{SoapySDRRange},
(Ptr{SoapySDRDevice}, Ptr{Csize_t}),
device,
length,
)
end
"""
SoapySDRDevice_listClockSources(device, length)
Get the list of available clock sources.
\\param device a pointer to a device instance
\\param [out] length the number of sources
\\return a list of clock source names
"""
function SoapySDRDevice_listClockSources(device, length)
@soapy_checked ccall(
(:SoapySDRDevice_listClockSources, soapysdr),
Ptr{Ptr{Cchar}},
(Ptr{SoapySDRDevice}, Ptr{Csize_t}),
device,
length,
)
end
"""
SoapySDRDevice_setClockSource(device, source)
Set the clock source on the device
\\param device a pointer to a device instance
\\param source the name of a clock source
\\return an error code or 0 for success
"""
function SoapySDRDevice_setClockSource(device, source)
@soapy_checked ccall(
(:SoapySDRDevice_setClockSource, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Ptr{Cchar}),
device,
source,
)
end
"""
SoapySDRDevice_getClockSource(device)
Get the clock source of the device
\\param device a pointer to a device instance
\\return the name of a clock source
"""
function SoapySDRDevice_getClockSource(device)
@soapy_checked ccall(
(:SoapySDRDevice_getClockSource, soapysdr),
Ptr{Cchar},
(Ptr{SoapySDRDevice},),
device,
)
end
"""
SoapySDRDevice_listTimeSources(device, length)
Get the list of available time sources.
\\param device a pointer to a device instance
\\param [out] length the number of sources
\\return a list of time source names
"""
function SoapySDRDevice_listTimeSources(device, length)
@soapy_checked ccall(
(:SoapySDRDevice_listTimeSources, soapysdr),
Ptr{Ptr{Cchar}},
(Ptr{SoapySDRDevice}, Ptr{Csize_t}),
device,
length,
)
end
"""
SoapySDRDevice_setTimeSource(device, source)
Set the time source on the device
\\param device a pointer to a device instance
\\param source the name of a time source
\\return an error code or 0 for success
"""
function SoapySDRDevice_setTimeSource(device, source)
@soapy_checked ccall(
(:SoapySDRDevice_setTimeSource, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Ptr{Cchar}),
device,
source,
)
end
"""
SoapySDRDevice_getTimeSource(device)
Get the time source of the device
\\param device a pointer to a device instance
\\return the name of a time source
"""
function SoapySDRDevice_getTimeSource(device)
@soapy_checked ccall(
(:SoapySDRDevice_getTimeSource, soapysdr),
Ptr{Cchar},
(Ptr{SoapySDRDevice},),
device,
)
end
"""
SoapySDRDevice_hasHardwareTime(device, what)
Does this device have a hardware clock?
\\param device a pointer to a device instance
\\param what optional argument
\\return true if the hardware clock exists
"""
function SoapySDRDevice_hasHardwareTime(device, what)
@soapy_checked ccall(
(:SoapySDRDevice_hasHardwareTime, soapysdr),
Bool,
(Ptr{SoapySDRDevice}, Ptr{Cchar}),
device,
what,
)
end
"""
SoapySDRDevice_getHardwareTime(device, what)
Read the time from the hardware clock on the device.
The what argument can refer to a specific time counter.
\\param device a pointer to a device instance
\\param what optional argument
\\return the time in nanoseconds
"""
function SoapySDRDevice_getHardwareTime(device, what)
@soapy_checked ccall(
(:SoapySDRDevice_getHardwareTime, soapysdr),
Clonglong,
(Ptr{SoapySDRDevice}, Ptr{Cchar}),
device,
what,
)
end
"""
SoapySDRDevice_setHardwareTime(device, timeNs, what)
Write the time to the hardware clock on the device.
The what argument can refer to a specific time counter.
\\param device a pointer to a device instance
\\param timeNs time in nanoseconds
\\param what optional argument
\\return 0 for success or error code on failure
"""
function SoapySDRDevice_setHardwareTime(device, timeNs, what)
@soapy_checked ccall(
(:SoapySDRDevice_setHardwareTime, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Clonglong, Ptr{Cchar}),
device,
timeNs,
what,
)
end
"""
SoapySDRDevice_setCommandTime(device, timeNs, what)
Set the time of subsequent configuration calls.
The what argument can refer to a specific command queue.
Implementations may use a time of 0 to clear.
\\deprecated replaced by setHardwareTime()
\\param device a pointer to a device instance
\\param timeNs time in nanoseconds
\\param what optional argument
\\return 0 for success or error code on failure
"""
function SoapySDRDevice_setCommandTime(device, timeNs, what)
@soapy_checked ccall(
(:SoapySDRDevice_setCommandTime, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Clonglong, Ptr{Cchar}),
device,
timeNs,
what,
)
end
"""
SoapySDRDevice_listSensors(device, length)
List the available global readback sensors.
A sensor can represent a reference lock, RSSI, temperature.
\\param device a pointer to a device instance
\\param [out] length the number of sensor names
\\return a list of available sensor string names
"""
function SoapySDRDevice_listSensors(device, length)
@soapy_checked ccall(
(:SoapySDRDevice_listSensors, soapysdr),
Ptr{Ptr{Cchar}},
(Ptr{SoapySDRDevice}, Ptr{Csize_t}),
device,
length,
)
end
"""
SoapySDRDevice_getSensorInfo(device, key)
Get meta-information about a sensor.
Example: displayable name, type, range.
\\param device a pointer to a device instance
\\param key the ID name of an available sensor
\\return meta-information about a sensor
"""
function SoapySDRDevice_getSensorInfo(device, key)
@soapy_checked ccall(
(:SoapySDRDevice_getSensorInfo, soapysdr),
SoapySDRArgInfo,
(Ptr{SoapySDRDevice}, Ptr{Cchar}),
device,
key,
)
end
"""
SoapySDRDevice_readSensor(device, key)
Readback a global sensor given the name.
The value returned is a string which can represent
a boolean ("true"/"false"), an integer, or float.
\\param device a pointer to a device instance
\\param key the ID name of an available sensor
\\return the current value of the sensor
"""
function SoapySDRDevice_readSensor(device, key)
@soapy_checked ccall(
(:SoapySDRDevice_readSensor, soapysdr),
Ptr{Cchar},
(Ptr{SoapySDRDevice}, Ptr{Cchar}),
device,
key,
)
end
"""
SoapySDRDevice_listChannelSensors(device, direction, channel, length)
List the available channel readback sensors.
A sensor can represent a reference lock, RSSI, temperature.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param [out] length the number of sensor names
\\return a list of available sensor string names
"""
function SoapySDRDevice_listChannelSensors(device, direction, channel, length)
@soapy_checked ccall(
(:SoapySDRDevice_listChannelSensors, soapysdr),
Ptr{Ptr{Cchar}},
(Ptr{SoapySDRDevice}, Cint, Csize_t, Ptr{Csize_t}),
device,
direction,
channel,
length,
)
end
"""
SoapySDRDevice_getChannelSensorInfo(device, direction, channel, key)
Get meta-information about a channel sensor.
Example: displayable name, type, range.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param key the ID name of an available sensor
\\return meta-information about a sensor
"""
function SoapySDRDevice_getChannelSensorInfo(device, direction, channel, key)
@soapy_checked ccall(
(:SoapySDRDevice_getChannelSensorInfo, soapysdr),
SoapySDRArgInfo,
(Ptr{SoapySDRDevice}, Cint, Csize_t, Ptr{Cchar}),
device,
direction,
channel,
key,
)
end
"""
SoapySDRDevice_readChannelSensor(device, direction, channel, key)
Readback a channel sensor given the name.
The value returned is a string which can represent
a boolean ("true"/"false"), an integer, or float.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param key the ID name of an available sensor
\\return the current value of the sensor
"""
function SoapySDRDevice_readChannelSensor(device, direction, channel, key)
@soapy_checked ccall(
(:SoapySDRDevice_readChannelSensor, soapysdr),
Ptr{Cchar},
(Ptr{SoapySDRDevice}, Cint, Csize_t, Ptr{Cchar}),
device,
direction,
channel,
key,
)
end
"""
SoapySDRDevice_listRegisterInterfaces(device, length)
Get a list of available register interfaces by name.
\\param device a pointer to a device instance
\\param [out] length the number of interfaces
\\return a list of available register interfaces
"""
function SoapySDRDevice_listRegisterInterfaces(device, length)
@soapy_checked ccall(
(:SoapySDRDevice_listRegisterInterfaces, soapysdr),
Ptr{Ptr{Cchar}},
(Ptr{SoapySDRDevice}, Ptr{Csize_t}),
device,
length,
)
end
"""
SoapySDRDevice_writeRegister(device, name, addr, value)
Write a register on the device given the interface name.
This can represent a register on a soft CPU, FPGA, IC;
the interpretation is up the implementation to decide.
\\param device a pointer to a device instance
\\param name the name of a available register interface
\\param addr the register address
\\param value the register value
\\return 0 for success or error code on failure
"""
function SoapySDRDevice_writeRegister(device, name, addr, value)
@soapy_checked ccall(
(:SoapySDRDevice_writeRegister, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Ptr{Cchar}, Cuint, Cuint),
device,
name,
addr,
value,
)
end
"""
SoapySDRDevice_readRegister(device, name, addr)
Read a register on the device given the interface name.
\\param device a pointer to a device instance
\\param name the name of a available register interface
\\param addr the register address
\\return the register value
"""
function SoapySDRDevice_readRegister(device, name, addr)
@soapy_checked ccall(
(:SoapySDRDevice_readRegister, soapysdr),
Cuint,
(Ptr{SoapySDRDevice}, Ptr{Cchar}, Cuint),
device,
name,
addr,
)
end
"""
SoapySDRDevice_writeRegisters(device, name, addr, value, length)
Write a memory block on the device given the interface name.
This can represent a memory block on a soft CPU, FPGA, IC;
the interpretation is up the implementation to decide.
\\param device a pointer to a device instance
\\param name the name of a available memory block interface
\\param addr the memory block start address
\\param value the memory block content
\\param length the number of words in the block
\\return 0 for success or error code on failure
"""
function SoapySDRDevice_writeRegisters(device, name, addr, value, length)
@soapy_checked ccall(
(:SoapySDRDevice_writeRegisters, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Ptr{Cchar}, Cuint, Ptr{Cuint}, Csize_t),
device,
name,
addr,
value,
length,
)
end
"""
SoapySDRDevice_readRegisters(device, name, addr, length)
Read a memory block on the device given the interface name.
Pass the number of words to be read in via length;
length will be set to the number of actual words read.
\\param device a pointer to a device instance
\\param name the name of a available memory block interface
\\param addr the memory block start address
\\param [inout] length number of words to be read from memory block
\\return the memory block content
"""
function SoapySDRDevice_readRegisters(device, name, addr, length)
@soapy_checked ccall(
(:SoapySDRDevice_readRegisters, soapysdr),
Ptr{Cuint},
(Ptr{SoapySDRDevice}, Ptr{Cchar}, Cuint, Ptr{Csize_t}),
device,
name,
addr,
length,
)
end
"""
SoapySDRDevice_getSettingInfo(device, length)
Describe the allowed keys and values used for settings.
\\param device a pointer to a device instance
\\param [out] length the number of sensor names
\\return a list of argument info structures
"""
function SoapySDRDevice_getSettingInfo(device, length)
@soapy_checked ccall(
(:SoapySDRDevice_getSettingInfo, soapysdr),
Ptr{SoapySDRArgInfo},
(Ptr{SoapySDRDevice}, Ptr{Csize_t}),
device,
length,
)
end
"""
SoapySDRDevice_writeSetting(device, key, value)
Write an arbitrary setting on the device.
The interpretation is up the implementation.
\\param device a pointer to a device instance
\\param key the setting identifier
\\param value the setting value
\\return 0 for success or error code on failure
"""
function SoapySDRDevice_writeSetting(device, key, value)
@soapy_checked ccall(
(:SoapySDRDevice_writeSetting, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Ptr{Cchar}, Ptr{Cchar}),
device,
key,
value,
)
end
"""
SoapySDRDevice_readSetting(device, key)
Read an arbitrary setting on the device.
\\param device a pointer to a device instance
\\param key the setting identifier
\\return the setting value
"""
function SoapySDRDevice_readSetting(device, key)
@soapy_checked ccall(
(:SoapySDRDevice_readSetting, soapysdr),
Ptr{Cchar},
(Ptr{SoapySDRDevice}, Ptr{Cchar}),
device,
key,
)
end
"""
SoapySDRDevice_getChannelSettingInfo(device, direction, channel, length)
Describe the allowed keys and values used for channel settings.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param [out] length the number of sensor names
\\return a list of argument info structures
"""
function SoapySDRDevice_getChannelSettingInfo(device, direction, channel, length)
@soapy_checked ccall(
(:SoapySDRDevice_getChannelSettingInfo, soapysdr),
Ptr{SoapySDRArgInfo},
(Ptr{SoapySDRDevice}, Cint, Csize_t, Ptr{Csize_t}),
device,
direction,
channel,
length,
)
end
"""
SoapySDRDevice_writeChannelSetting(device, direction, channel, key, value)
Write an arbitrary channel setting on the device.
The interpretation is up the implementation.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param key the setting identifier
\\param value the setting value
\\return 0 for success or error code on failure
"""
function SoapySDRDevice_writeChannelSetting(device, direction, channel, key, value)
@soapy_checked ccall(
(:SoapySDRDevice_writeChannelSetting, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Cint, Csize_t, Ptr{Cchar}, Ptr{Cchar}),
device,
direction,
channel,
key,
value,
)
end
"""
SoapySDRDevice_readChannelSetting(device, direction, channel, key)
Read an arbitrary channel setting on the device.
\\param device a pointer to a device instance
\\param direction the channel direction RX or TX
\\param channel an available channel on the device
\\param key the setting identifier
\\return the setting value
"""
function SoapySDRDevice_readChannelSetting(device, direction, channel, key)
@soapy_checked ccall(
(:SoapySDRDevice_readChannelSetting, soapysdr),
Ptr{Cchar},
(Ptr{SoapySDRDevice}, Cint, Csize_t, Ptr{Cchar}),
device,
direction,
channel,
key,
)
end
"""
SoapySDRDevice_listGPIOBanks(device, length)
Get a list of available GPIO banks by name.
\\param [out] length the number of GPIO banks
\\param device a pointer to a device instance
"""
function SoapySDRDevice_listGPIOBanks(device, length)
@soapy_checked ccall(
(:SoapySDRDevice_listGPIOBanks, soapysdr),
Ptr{Ptr{Cchar}},
(Ptr{SoapySDRDevice}, Ptr{Csize_t}),
device,
length,
)
end
"""
SoapySDRDevice_writeGPIO(device, bank, value)
Write the value of a GPIO bank.
\\param device a pointer to a device instance
\\param bank the name of an available bank
\\param value an integer representing GPIO bits
\\return 0 for success or error code on failure
"""
function SoapySDRDevice_writeGPIO(device, bank, value)
@soapy_checked ccall(
(:SoapySDRDevice_writeGPIO, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Ptr{Cchar}, Cuint),
device,
bank,
value,
)
end
"""
SoapySDRDevice_writeGPIOMasked(device, bank, value, mask)
Write the value of a GPIO bank with modification mask.
\\param device a pointer to a device instance
\\param bank the name of an available bank
\\param value an integer representing GPIO bits
\\param mask a modification mask where 1 = modify
\\return 0 for success or error code on failure
"""
function SoapySDRDevice_writeGPIOMasked(device, bank, value, mask)
@soapy_checked ccall(
(:SoapySDRDevice_writeGPIOMasked, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Ptr{Cchar}, Cuint, Cuint),
device,
bank,
value,
mask,
)
end
"""
SoapySDRDevice_readGPIO(device, bank)
Readback the value of a GPIO bank.
\\param device a pointer to a device instance
\\param bank the name of an available bank
\\return an integer representing GPIO bits
"""
function SoapySDRDevice_readGPIO(device, bank)
@soapy_checked ccall(
(:SoapySDRDevice_readGPIO, soapysdr),
Cuint,
(Ptr{SoapySDRDevice}, Ptr{Cchar}),
device,
bank,
)
end
"""
SoapySDRDevice_writeGPIODir(device, bank, dir)
Write the data direction of a GPIO bank.
1 bits represent outputs, 0 bits represent inputs.
\\param device a pointer to a device instance
\\param bank the name of an available bank
\\param dir an integer representing data direction bits
\\return 0 for success or error code on failure
"""
function SoapySDRDevice_writeGPIODir(device, bank, dir)
@soapy_checked ccall(
(:SoapySDRDevice_writeGPIODir, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Ptr{Cchar}, Cuint),
device,
bank,
dir,
)
end
"""
SoapySDRDevice_writeGPIODirMasked(device, bank, dir, mask)
Write the data direction of a GPIO bank with modification mask.
1 bits represent outputs, 0 bits represent inputs.
\\param device a pointer to a device instance
\\param bank the name of an available bank
\\param dir an integer representing data direction bits
\\param mask a modification mask where 1 = modify
\\return 0 for success or error code on failure
"""
function SoapySDRDevice_writeGPIODirMasked(device, bank, dir, mask)
@soapy_checked ccall(
(:SoapySDRDevice_writeGPIODirMasked, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Ptr{Cchar}, Cuint, Cuint),
device,
bank,
dir,
mask,
)
end
"""
SoapySDRDevice_readGPIODir(device, bank)
Read the data direction of a GPIO bank.
\\param device a pointer to a device instance
1 bits represent outputs, 0 bits represent inputs.
\\param bank the name of an available bank
\\return an integer representing data direction bits
"""
function SoapySDRDevice_readGPIODir(device, bank)
@soapy_checked ccall(
(:SoapySDRDevice_readGPIODir, soapysdr),
Cuint,
(Ptr{SoapySDRDevice}, Ptr{Cchar}),
device,
bank,
)
end
"""
SoapySDRDevice_writeI2C(device, addr, data, numBytes)
Write to an available I2C slave.
If the device contains multiple I2C masters,
the address bits can encode which master.
\\param device a pointer to a device instance
\\param addr the address of the slave
\\param data an array of bytes write out
\\param numBytes the number of bytes to write
\\return 0 for success or error code on failure
"""
function SoapySDRDevice_writeI2C(device, addr, data, numBytes)
@soapy_checked ccall(
(:SoapySDRDevice_writeI2C, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Cint, Ptr{Cchar}, Csize_t),
device,
addr,
data,
numBytes,
)
end
"""
SoapySDRDevice_readI2C(device, addr, numBytes)
Read from an available I2C slave.
If the device contains multiple I2C masters,
the address bits can encode which master.
Pass the number of bytes to be read in via numBytes;
numBytes will be set to the number of actual bytes read.
\\param device a pointer to a device instance
\\param addr the address of the slave
\\param [inout] numBytes the number of bytes to read
\\return an array of bytes read from the slave
"""
function SoapySDRDevice_readI2C(device, addr, numBytes)
@soapy_checked ccall(
(:SoapySDRDevice_readI2C, soapysdr),
Ptr{Cchar},
(Ptr{SoapySDRDevice}, Cint, Ptr{Csize_t}),
device,
addr,
numBytes,
)
end
"""
SoapySDRDevice_transactSPI(device, addr, data, numBits)
Perform a SPI transaction and return the result.
Its up to the implementation to set the clock rate,
and read edge, and the write edge of the SPI core.
SPI slaves without a readback pin will return 0.
If the device contains multiple SPI masters,
the address bits can encode which master.
\\param device a pointer to a device instance
\\param addr an address of an available SPI slave
\\param data the SPI data, numBits-1 is first out
\\param numBits the number of bits to clock out
\\return the readback data, numBits-1 is first in
"""
function SoapySDRDevice_transactSPI(device, addr, data, numBits)
@soapy_checked ccall(
(:SoapySDRDevice_transactSPI, soapysdr),
Cuint,
(Ptr{SoapySDRDevice}, Cint, Cuint, Csize_t),
device,
addr,
data,
numBits,
)
end
"""
SoapySDRDevice_listUARTs(device, length)
Enumerate the available UART devices.
\\param device a pointer to a device instance
\\param [out] length the number of UART names
\\return a list of names of available UARTs
"""
function SoapySDRDevice_listUARTs(device, length)
@soapy_checked ccall(
(:SoapySDRDevice_listUARTs, soapysdr),
Ptr{Ptr{Cchar}},
(Ptr{SoapySDRDevice}, Ptr{Csize_t}),
device,
length,
)
end
"""
SoapySDRDevice_writeUART(device, which, data)
Write data to a UART device.
Its up to the implementation to set the baud rate,
carriage return settings, flushing on newline.
\\param device a pointer to a device instance
\\param which the name of an available UART
\\param data a null terminated array of bytes
\\return 0 for success or error code on failure
"""
function SoapySDRDevice_writeUART(device, which, data)
@soapy_checked ccall(
(:SoapySDRDevice_writeUART, soapysdr),
Cint,
(Ptr{SoapySDRDevice}, Ptr{Cchar}, Ptr{Cchar}),
device,
which,
data,
)
end
"""
SoapySDRDevice_readUART(device, which, timeoutUs)
Read bytes from a UART until timeout or newline.
Its up to the implementation to set the baud rate,
carriage return settings, flushing on newline.
\\param device a pointer to a device instance
\\param which the name of an available UART
\\param timeoutUs a timeout in microseconds
\\return a null terminated array of bytes
"""
function SoapySDRDevice_readUART(device, which, timeoutUs)
@soapy_checked ccall(
(:SoapySDRDevice_readUART, soapysdr),
Ptr{Cchar},
(Ptr{SoapySDRDevice}, Ptr{Cchar}, Clong),
device,
which,
timeoutUs,
)
end
"""
SoapySDRDevice_getNativeDeviceHandle(device)
A handle to the native device used by the driver.
The implementation may return a null value if it does not support
or does not wish to provide access to the native handle.
\\param device a pointer to a device instance
\\return a handle to the native device or null
"""
function SoapySDRDevice_getNativeDeviceHandle(device)
@soapy_checked ccall(
(:SoapySDRDevice_getNativeDeviceHandle, soapysdr),
Ptr{Cvoid},
(Ptr{SoapySDRDevice},),
device,
)
end
"""
SoapySDR_errToStr(errorCode)
Convert a error code to a string for printing purposes.
If the error code is unrecognized, errToStr returns "UNKNOWN".
\\param errorCode a negative integer return code
\\return a pointer to a string representing the error
"""
function SoapySDR_errToStr(errorCode)
ccall((:SoapySDR_errToStr, soapysdr), Ptr{Cchar}, (Cint,), errorCode)
end
"""
SoapySDR_formatToSize(format)
Get the size of a single element in the specified format.
\\param format a supported format string
\\return the size of an element in bytes
"""
function SoapySDR_formatToSize(format)
ccall((:SoapySDR_formatToSize, soapysdr), Csize_t, (Ptr{Cchar},), format)
end
"""
SoapySDRLogLevel
The available priority levels for log messages.
The default log level threshold is SOAPY_SDR_INFO.
Log messages with lower priorities are dropped.
The default threshold can be set via the
SOAPY_SDR_LOG_LEVEL environment variable.
Set SOAPY_SDR_LOG_LEVEL to the string value:
"WARNING", "ERROR", "DEBUG", etc...
or set it to the equivalent integer value.
"""
@cenum SoapySDRLogLevel::UInt32 begin
SOAPY_SDR_FATAL = 1
SOAPY_SDR_CRITICAL = 2
SOAPY_SDR_ERROR = 3
SOAPY_SDR_WARNING = 4
SOAPY_SDR_NOTICE = 5
SOAPY_SDR_INFO = 6
SOAPY_SDR_DEBUG = 7
SOAPY_SDR_TRACE = 8
SOAPY_SDR_SSI = 9
end
"""
SoapySDR_log(logLevel, message)
Send a message to the registered logger.
\\param logLevel a possible logging level
\\param message a logger message string
"""
function SoapySDR_log(logLevel, message)
ccall(
(:SoapySDR_log, soapysdr),
Cvoid,
(SoapySDRLogLevel, Ptr{Cchar}),
logLevel,
message,
)
end
# typedef void ( * SoapySDRLogHandler ) ( const SoapySDRLogLevel logLevel , const char * message )
"""
Typedef for the registered log handler function.
"""
const SoapySDRLogHandler = Ptr{Cvoid}
"""
SoapySDR_registerLogHandler(handler)
Register a new system log handler.
Platforms should call this to replace the default stdio handler.
Passing `NULL` restores the default.
"""
function SoapySDR_registerLogHandler(handler)
ccall((:SoapySDR_registerLogHandler, soapysdr), Cvoid, (SoapySDRLogHandler,), handler)
end
"""
SoapySDR_setLogLevel(logLevel)
Set the log level threshold.
Log messages with lower priority are dropped.
"""
function SoapySDR_setLogLevel(logLevel)
ccall((:SoapySDR_setLogLevel, soapysdr), Cvoid, (SoapySDRLogLevel,), logLevel)
end
"""
SoapySDR_getRootPath()
Query the root installation path
"""
function SoapySDR_getRootPath()
ccall((:SoapySDR_getRootPath, soapysdr), Ptr{Cchar}, ())
end
"""
SoapySDR_listSearchPaths(length)
The list of paths automatically searched by loadModules().
\\param [out] length the number of elements in the result.
\\return a list of automatically searched file paths
"""
function SoapySDR_listSearchPaths(length)
ccall((:SoapySDR_listSearchPaths, soapysdr), Ptr{Ptr{Cchar}}, (Ptr{Csize_t},), length)
end
"""
SoapySDR_listModules(length)
List all modules found in default path.
The result is an array of strings owned by the caller.
\\param [out] length the number of elements in the result.
\\return a list of file paths to loadable modules
"""
function SoapySDR_listModules(length)
ccall((:SoapySDR_listModules, soapysdr), Ptr{Ptr{Cchar}}, (Ptr{Csize_t},), length)
end
"""
SoapySDR_listModulesPath(path, length)
List all modules found in the given path.
The result is an array of strings owned by the caller.
\\param path a directory on the system
\\param [out] length the number of elements in the result.
\\return a list of file paths to loadable modules
"""
function SoapySDR_listModulesPath(path, length)
ccall(
(:SoapySDR_listModulesPath, soapysdr),
Ptr{Ptr{Cchar}},
(Ptr{Cchar}, Ptr{Csize_t}),
path,
length,
)
end
"""
SoapySDR_loadModule(path)
Load a single module given its file system path.
The caller must free the result error string.
\\param path the path to a specific module file
\\return an error message, empty on success
"""
function SoapySDR_loadModule(path)
ccall((:SoapySDR_loadModule, soapysdr), Ptr{Cchar}, (Ptr{Cchar},), path)
end
"""
SoapySDR_getLoaderResult(path)
List all registration loader errors for a given module path.
The resulting dictionary contains all registry entry names
provided by the specified module. The value of each entry
is an error message string or empty on successful load.
\\param path the path to a specific module file
\\return a dictionary of registry names to error messages
"""
function SoapySDR_getLoaderResult(path)
ccall((:SoapySDR_getLoaderResult, soapysdr), SoapySDRKwargs, (Ptr{Cchar},), path)
end
"""
SoapySDR_getModuleVersion(path)
Get a version string for the specified module.
Modules may optionally provide version strings.
\\param path the path to a specific module file
\\return a version string or empty if no version provided
"""
function SoapySDR_getModuleVersion(path)
ccall((:SoapySDR_getModuleVersion, soapysdr), Ptr{Cchar}, (Ptr{Cchar},), path)
end
"""
SoapySDR_unloadModule(path)
Unload a module that was loaded with loadModule().
The caller must free the result error string.
\\param path the path to a specific module file
\\return an error message, empty on success
"""
function SoapySDR_unloadModule(path)
ccall((:SoapySDR_unloadModule, soapysdr), Ptr{Cchar}, (Ptr{Cchar},), path)
end
"""
SoapySDR_loadModules()
Load the support modules installed on this system.
This call will only actually perform the load once.
Subsequent calls are a NOP.
"""
function SoapySDR_loadModules()
ccall((:SoapySDR_loadModules, soapysdr), Cvoid, ())
end
"""
SoapySDR_unloadModules()
Unload all currently loaded support modules.
"""
function SoapySDR_unloadModules()
ccall((:SoapySDR_unloadModules, soapysdr), Cvoid, ())
end
"""
SoapySDR_ticksToTimeNs(ticks, rate)
Convert a tick count into a time in nanoseconds using the tick rate.
\\param ticks a integer tick count
\\param rate the ticks per second
\\return the time in nanoseconds
"""
function SoapySDR_ticksToTimeNs(ticks, rate)
ccall((:SoapySDR_ticksToTimeNs, soapysdr), Clonglong, (Clonglong, Cdouble), ticks, rate)
end
"""
SoapySDR_timeNsToTicks(timeNs, rate)
Convert a time in nanoseconds into a tick count using the tick rate.
\\param timeNs time in nanoseconds
\\param rate the ticks per second
\\return the integer tick count
"""
function SoapySDR_timeNsToTicks(timeNs, rate)
ccall(
(:SoapySDR_timeNsToTicks, soapysdr),
Clonglong,
(Clonglong, Cdouble),
timeNs,
rate,
)
end
"""
SoapySDRKwargs_fromString(markup)
Convert a markup string to a key-value map.
The markup format is: "key0=value0, key1=value1"
"""
function SoapySDRKwargs_fromString(markup)
ccall((:SoapySDRKwargs_fromString, soapysdr), SoapySDRKwargs, (Ptr{Cchar},), markup)
end
"""
SoapySDRKwargs_toString(args)
Convert a key-value map to a markup string.
The markup format is: "key0=value0, key1=value1"
"""
function SoapySDRKwargs_toString(args)
ccall((:SoapySDRKwargs_toString, soapysdr), Ptr{Cchar}, (Ptr{SoapySDRKwargs},), args)
end
"""
SoapySDR_free(ptr)
Free a pointer allocated by SoapySDR.
For most platforms this is a simple call around free()
"""
function SoapySDR_free(ptr)
ccall((:SoapySDR_free, soapysdr), Cvoid, (Ptr{Cvoid},), ptr)
end
"""
SoapySDRStrings_clear(elems, length)
Clear the contents of a list of string
Convenience call to deal with results that return a string list.
"""
function SoapySDRStrings_clear(elems, length)
ccall(
(:SoapySDRStrings_clear, soapysdr),
Cvoid,
(Ptr{Ptr{Ptr{Cchar}}}, Csize_t),
elems,
length,
)
end
"""
SoapySDRKwargs_set(args, key, val)
Set a key/value pair in a kwargs structure.
\\post
If the key exists, the existing entry will be modified;
otherwise a new entry will be appended to args.
On error, the elements of args will not be modified,
and args is guaranteed to be in a good state.
\\return 0 for success, otherwise allocation error
"""
function SoapySDRKwargs_set(args, key, val)
ccall(
(:SoapySDRKwargs_set, soapysdr),
Cint,
(Ptr{SoapySDRKwargs}, Ptr{Cchar}, Ptr{Cchar}),
args,
key,
val,
)
end
"""
SoapySDRKwargs_get(args, key)
Get a value given a key in a kwargs structure.
\\return the string or NULL if not found
"""
function SoapySDRKwargs_get(args, key)
ccall(
(:SoapySDRKwargs_get, soapysdr),
Ptr{Cchar},
(Ptr{SoapySDRKwargs}, Ptr{Cchar}),
args,
key,
)
end
"""
SoapySDRKwargs_clear(args)
Clear the contents of a kwargs structure.
This frees all the underlying memory and clears the members.
"""
function SoapySDRKwargs_clear(args)
ccall((:SoapySDRKwargs_clear, soapysdr), Cvoid, (Ptr{SoapySDRKwargs},), args)
end
"""
SoapySDRKwargsList_clear(args, length)
Clear a list of kwargs structures.
This frees all the underlying memory and clears the members.
"""
function SoapySDRKwargsList_clear(args, length)
ccall(
(:SoapySDRKwargsList_clear, soapysdr),
Cvoid,
(Ptr{SoapySDRKwargs}, Csize_t),
args,
length,
)
end
"""
SoapySDRArgInfo_clear(info)
Clear the contents of a argument info structure.
This frees all the underlying memory and clears the members.
"""
function SoapySDRArgInfo_clear(info)
ccall((:SoapySDRArgInfo_clear, soapysdr), Cvoid, (Ptr{SoapySDRArgInfo},), info)
end
"""
SoapySDRArgInfoList_clear(info, length)
Clear a list of argument info structures.
This frees all the underlying memory and clears the members.
"""
function SoapySDRArgInfoList_clear(info, length)
ccall(
(:SoapySDRArgInfoList_clear, soapysdr),
Cvoid,
(Ptr{SoapySDRArgInfo}, Csize_t),
info,
length,
)
end
"""
SoapySDR_getAPIVersion()
Get the SoapySDR library API version as a string.
The format of the version string is <b>major.minor.increment</b>,
where the digits are taken directly from <b>SOAPY_SDR_API_VERSION</b>.
"""
function SoapySDR_getAPIVersion()
ccall((:SoapySDR_getAPIVersion, soapysdr), Ptr{Cchar}, ())
end
"""
SoapySDR_getABIVersion()
Get the ABI version string that the library was built against.
A client can compare <b>SOAPY_SDR_ABI_VERSION</b> to getABIVersion()
to check for ABI incompatibility before using the library.
If the values are not equal then the client code was
compiled against a different ABI than the library.
"""
function SoapySDR_getABIVersion()
ccall((:SoapySDR_getABIVersion, soapysdr), Ptr{Cchar}, ())
end
"""
SoapySDR_getLibVersion()
Get the library version and build information string.
The format of the version string is <b>major.minor.patch-buildInfo</b>.
This function is commonly used to identify the software back-end
to the user for command-line utilities and graphical applications.
"""
function SoapySDR_getLibVersion()
ccall((:SoapySDR_getLibVersion, soapysdr), Ptr{Cchar}, ())
end
# Skipping MacroDefinition: SOAPY_SDR_HELPER_DLL_IMPORT __attribute__ ( ( visibility ( "default" ) ) )
# Skipping MacroDefinition: SOAPY_SDR_HELPER_DLL_EXPORT __attribute__ ( ( visibility ( "default" ) ) )
# Skipping MacroDefinition: SOAPY_SDR_HELPER_DLL_LOCAL __attribute__ ( ( visibility ( "hidden" ) ) )
# Skipping MacroDefinition: SOAPY_SDR_EXTERN extern
const SOAPY_SDR_TX = 0
const SOAPY_SDR_RX = 1
const SOAPY_SDR_END_BURST = 1 << 1
const SOAPY_SDR_HAS_TIME = 1 << 2
const SOAPY_SDR_END_ABRUPT = 1 << 3
const SOAPY_SDR_ONE_PACKET = 1 << 4
const SOAPY_SDR_MORE_FRAGMENTS = 1 << 5
const SOAPY_SDR_WAIT_TRIGGER = 1 << 6
const SOAPY_SDR_TIMEOUT = -1
const SOAPY_SDR_STREAM_ERROR = -2
const SOAPY_SDR_CORRUPTION = -3
const SOAPY_SDR_OVERFLOW = -4
const SOAPY_SDR_NOT_SUPPORTED = -5
const SOAPY_SDR_TIME_ERROR = -6
const SOAPY_SDR_UNDERFLOW = -7
const SOAPY_SDR_CF64 = "CF64"
const SOAPY_SDR_CF32 = "CF32"
const SOAPY_SDR_CS32 = "CS32"
const SOAPY_SDR_CU32 = "CU32"
const SOAPY_SDR_CS16 = "CS16"
const SOAPY_SDR_CU16 = "CU16"
const SOAPY_SDR_CS12 = "CS12"
const SOAPY_SDR_CU12 = "CU12"
const SOAPY_SDR_CS8 = "CS8"
const SOAPY_SDR_CU8 = "CU8"
const SOAPY_SDR_CS4 = "CS4"
const SOAPY_SDR_CU4 = "CU4"
const SOAPY_SDR_F64 = "F64"
const SOAPY_SDR_F32 = "F32"
const SOAPY_SDR_S32 = "S32"
const SOAPY_SDR_U32 = "U32"
const SOAPY_SDR_S16 = "S16"
const SOAPY_SDR_U16 = "U16"
const SOAPY_SDR_S8 = "S8"
const SOAPY_SDR_U8 = "U8"
const SOAPY_SDR_SSI = SOAPY_SDR_SSI
const SOAPY_SDR_TRUE = "true"
const SOAPY_SDR_FALSE = "false"
const SOAPY_SDR_API_VERSION = 0x00080000
const SOAPY_SDR_ABI_VERSION = "0.8"
| SoapySDR | https://github.com/JuliaTelecom/SoapySDR.jl.git |
|
[
"MIT",
"BSL-1.0"
] | 0.5.0 | e96812c38a7ca1c013d35c9515f05a1d023e2191 | code | 9567 | using SoapySDR
using Test
using Unitful
using Unitful.DefaultSymbols
using Intervals
const dB = u"dB"
const sd = SoapySDR
const hardware = "loopback"
# Load dummy test harness or hardware
if hardware == "loopback"
using SoapyLoopback_jll
elseif hardware == "rtlsdr"
using SoapyRTLSDR_jll
else
error("unknown test hardware")
end
# Test Log Handler registration
SoapySDR.register_log_handler()
@testset "SoapySDR.jl" begin
@testset "Version" begin
SoapySDR.versioninfo()
end
@testset "Logging" begin
SoapySDR.register_log_handler()
SoapySDR.set_log_level(0)
end
@testset "Error" begin
SoapySDR.error_to_string(-1) == "TIMEOUT"
SoapySDR.error_to_string(10) == "UNKNOWN"
end
@testset "Ranges/Display" begin
intervalrange = sd.SoapySDRRange(0, 1, 0)
steprange = sd.SoapySDRRange(0, 1, 0.1)
intervalrangedb = sd._gainrange(intervalrange)
steprangedb = sd._gainrange(steprange) #TODO
intervalrangehz = sd._hzrange(intervalrange)
steprangehz = sd._hzrange(steprange)
hztype = typeof(1.0 * Hz)
@test typeof(intervalrangedb) ==
Interval{Gain{Unitful.LogInfo{:Decibel,10,10},:?,Float64},Closed,Closed}
@test typeof(steprangedb) ==
Interval{Gain{Unitful.LogInfo{:Decibel,10,10},:?,Float64},Closed,Closed}
@test typeof(intervalrangehz) == Interval{hztype,Closed,Closed}
if VERSION >= v"1.7"
@test typeof(steprangehz) == StepRangeLen{
hztype,
Base.TwicePrecision{hztype},
Base.TwicePrecision{hztype},
Int64,
}
else
@test typeof(steprangehz) == StepRangeLen{
hztype,
Base.TwicePrecision{hztype},
Base.TwicePrecision{hztype},
}
end
io = IOBuffer(read = true, write = true)
sd.print_hz_range(io, intervalrangehz)
@test String(take!(io)) == "00..0.001 kHz"
sd.print_hz_range(io, steprangehz)
@test String(take!(io)) == "00 Hz:0.0001 kHz:0.001 kHz"
end
@testset "Keyword Arguments" begin
args = KWArgs()
@test length(args) == 0
args = parse(KWArgs, "foo=1,bar=2")
@test length(args) == 2
@test args["foo"] == "1"
@test args["bar"] == "2"
args["foo"] = "0"
@test args["foo"] == "0"
args["qux"] = "3"
@test length(args) == 3
@test args["qux"] == "3"
str = String(args)
@test contains(str, "foo=0")
end
@testset "High Level API" begin
io = IOBuffer(read = true, write = true)
# Test failing to open a device due to an invalid specification
@test_throws SoapySDR.SoapySDRDeviceError Device(parse(KWArgs, "driver=foo"))
# Device constructor, show, iterator
@test length(Devices()) == 1
@test length(Devices(driver = "loopback")) == 1
show(io, Devices())
deva = Devices()[1]
deva["refclk"] = "internal"
dev = Device(deva)
show(io, dev)
for dev in Devices()
show(io, dev)
end
@test typeof(dev) == sd.Device
@test typeof(dev.info) == sd.KWArgs
@test dev.driver == :LoopbackDriver
@test dev.hardware == :LoopbackHardware
@test dev.time_sources == SoapySDR.TimeSource[:sw_ticks, :hw_ticks]
@test dev.time_source == SoapySDR.TimeSource(:sw_ticks)
dev.time_source = "hw_ticks"
@test dev.time_source == SoapySDR.TimeSource(:hw_ticks)
dev.time_source = dev.time_sources[1]
@test dev.time_source == SoapySDR.TimeSource(:sw_ticks)
# Channels
rx_chan_list = dev.rx
tx_chan_list = dev.tx
@test typeof(rx_chan_list) == sd.ChannelList
@test typeof(tx_chan_list) == sd.ChannelList
show(io, rx_chan_list)
show(io, tx_chan_list)
rx_chan = dev.rx[1]
tx_chan = dev.tx[1]
@test typeof(rx_chan) == sd.Channel
@test typeof(tx_chan) == sd.Channel
show(io, rx_chan)
show(io, tx_chan)
show(io, MIME"text/plain"(), rx_chan)
show(io, MIME"text/plain"(), tx_chan)
#channel set/get properties
@test rx_chan.native_stream_format == SoapySDR.ComplexInt{12} #, fullscale
@test rx_chan.stream_formats ==
[Complex{Int8}, SoapySDR.ComplexInt{12}, Complex{Int16}, ComplexF32]
@test tx_chan.native_stream_format == SoapySDR.ComplexInt{12} #, fullscale
@test tx_chan.stream_formats ==
[Complex{Int8}, SoapySDR.ComplexInt{12}, Complex{Int16}, ComplexF32]
@test rx_chan.antennas == SoapySDR.Antenna[:RX, :TX]
@test tx_chan.antennas == SoapySDR.Antenna[:RX, :TX]
@test rx_chan.antenna == SoapySDR.Antenna(:RX)
@test tx_chan.antenna == SoapySDR.Antenna(:TX)
rx_chan.antenna = :TX
# TODO: Add some more antennas to the loopback driver
#@test rx_chan.antenna == SoapySDR.Antenna(:TX)
rx_chan.antenna = :RX
rx_chan.antenna = "RX"
@test_throws ArgumentError rx_chan.antenna = 100
@test rx_chan.antenna == SoapySDR.Antenna(:RX)
# channel gain tests
@test rx_chan.gain_mode == false
rx_chan.gain_mode = true
@test rx_chan.gain_mode == true
@test rx_chan.gain_elements ==
SoapySDR.GainElement[:IF1, :IF2, :IF3, :IF4, :IF5, :IF6, :TUNER]
if1 = rx_chan.gain_elements[1]
@show rx_chan[if1]
rx_chan[if1] = 0.5u"dB"
@test rx_chan[if1] == 0.5u"dB"
#@show rx_chan.gain_profile
@show rx_chan.frequency_correction
#@test tx_chan.bandwidth == 2.048e6u"Hz"
#@test tx_chan.frequency == 1.0e8u"Hz"
#@test tx_chan.gain == -53u"dB"
#@test tx_chan.sample_rate == 2.048e6u"Hz"
# setter/getter tests
rx_chan.sample_rate = 1e5u"Hz"
#@test rx_chan.sample_rate == 1e5u"Hz"
@test_logs (:warn, r"poorly supported") match_mode = :any begin
rx_stream = sd.Stream([rx_chan])
@test typeof(rx_stream) == sd.Stream{sd.ComplexInt{12}}
tx_stream = sd.Stream([tx_chan])
@test typeof(tx_stream) == sd.Stream{sd.ComplexInt{12}}
@test tx_stream.nchannels == 1
@test rx_stream.nchannels == 1
@test tx_stream.num_direct_access_buffers == 0x000000000000000f
@test tx_stream.mtu == 0x0000000000020000
@test rx_stream.num_direct_access_buffers == 0x000000000000000f
@test rx_stream.mtu == 0x0000000000020000
end
rx_stream = sd.Stream(ComplexF32, [rx_chan])
@test typeof(rx_stream) == sd.Stream{ComplexF32}
tx_stream = sd.Stream(ComplexF32, [tx_chan])
@test typeof(tx_stream) == sd.Stream{ComplexF32}
sd.activate!(rx_stream)
sd.activate!(tx_stream)
# First, try to write an invalid buffer type, ensure that that errors
buffers = [zeros(ComplexF32, 10), zeros(ComplexF32, 11)]
@test_throws ArgumentError write(tx_stream, buffers)
# We (unfortunately) cannot do a write/read test, as that's not supported
# by SoapyLoopback yet, despite the name. ;)
#=
tx_buff = randn(ComplexF32, tx_stream.mtu)
write(tx_stream, (tx_buff,))
rx_buff = vcat(
only(read(rx_stream, div(rx_stream.mtu,2))),
only(read(rx_stream, div(rx_stream.mtu,2))),
)
@test length(rx_buff) == length(tx_buff)
@test all(rx_buff .== tx_buff)
=#
# Test that reading once more causes a warning to be printed, as there's nothing to be read:
@test_logs (:warn, r"readStream timeout!") match_mode = :any begin
read(rx_stream, 1)
end
sd.deactivate!(rx_stream)
sd.deactivate!(tx_stream)
# do block syntax
Device(Devices()[1]) do dev
println(dev.info)
end
# and again to ensure correct GC
Device(Devices()[1]) do dev
sd.Stream(ComplexF32, [dev.rx[1]]) do s_rx
println(dev.info)
println(s_rx)
# Activate/deactivate
sd.activate!(s_rx) do
end
# Group activate/deactivate
sd.Stream(ComplexF32, [dev.tx[1]]) do s_tx
sd.activate!([s_rx, s_tx]) do
end
end
end
end
end
@testset "Settings" begin
io = IOBuffer(read = true, write = true)
dev = Device(Devices()[1])
arglist = SoapySDR.ArgInfoList(SoapySDR.SoapySDRDevice_getSettingInfo(dev)...)
println(arglist)
a1 = arglist[1]
println(a1)
end
@testset "Examples" begin
include("../examples/highlevel_dump_devices.jl")
end
@testset "Modules" begin
@test SoapySDR.Modules.get_root_path() == "/workspace/destdir"
@test all(
SoapySDR.Modules.list_search_paths() .==
["/workspace/destdir/lib/SoapySDR/modules0.8"],
)
@test SoapySDR.Modules.list() == String[]
end
using Aqua
# Aqua tests
# Intervals brings a bunch of ambiquities unfortunately
Aqua.test_all(SoapySDR; ambiguities = false)
end #SoapySDR testset
if VERSION >= v"1.8"
@info "Running JET..."
using JET
display(JET.report_package(SoapySDR))
end
| SoapySDR | https://github.com/JuliaTelecom/SoapySDR.jl.git |
|
[
"MIT",
"BSL-1.0"
] | 0.5.0 | e96812c38a7ca1c013d35c9515f05a1d023e2191 | docs | 913 | # SoapySDR.jl
[](https://juliatelecom.github.io/SoapySDR.jl/dev/)
[](https://coveralls.io/github/JuliaTelecom/SoapySDR.jl?branch=main)
A Julia wrapper for [SoapySDR](https://github.com/pothosware/SoapySDR/wiki) to enable SDR processing in Julia.
It features a high level interface to interact with underlying SDR radios.
Several radios are supported in the Julia Package manager, and may be easily installed for use with SoapySDR.jl
- [Quick Start](https://juliatelecom.github.io/SoapySDR.jl/dev/#Quick-Start)
- [Tutorial](https://juliatelecom.github.io/SoapySDR.jl/dev/tutorial/)
- [High Level API](https://juliatelecom.github.io/SoapySDR.jl/dev/highlevel/)
- [Supported Radios](https://juliatelecom.github.io/SoapySDR.jl/dev/#Loading-a-Driver-Module)
| SoapySDR | https://github.com/JuliaTelecom/SoapySDR.jl.git |
|
[
"MIT",
"BSL-1.0"
] | 0.5.0 | e96812c38a7ca1c013d35c9515f05a1d023e2191 | docs | 1480 | # Loading Drivers
Below is a list of driver modules that may be installed with the Julia package manager.
| Device | Julia Package |
|---------|------------------|
| xrtx | xtrx_jll |
|RTL-SDR | SoapyRTLSDR_jll |
|LimeSDR | SoapyLMS7_jll |
| USRP | SoapyUHD_jll |
|Pluto SDR| SoapyPlutoSDR_jll|
If you need a driver module that is not listed, you can search [JuliaHub](https://juliahub.com)
to see if it may have been added to the package manager. If not, please file an [issue](https://github.com/JuliaTelecom/SoapySDR.jl/issues).
Alternatively, you can see the instructions below about using operating system provided modules with SoapySDR.jl.
To activate the driver and module, simply use the package along with SoapySDR.
For example:
```
julia> using SoapySDR, xtrx_jll
```
or:
```
julia> using SoapySDR, SoapyRTLSDR_jll
```
## Loading System-Provided Driver Modules
The `SOAPY_SDR_PLUGIN_PATH` environmental variable is read by SoapySDR to load local driver modules.
For example, on Ubuntu one may use the Ubuntu package manager to install all SoapySDR driver modules:
```
sudo apt install soapysdr0.8-module-all
```
These can then be used from SoapySDR.jl by exporting the environmental variable with the module directory:
```
export SOAPY_SDR_PLUGIN_PATH=/usr/lib/x86_64-linux-gnu/SoapySDR/modules0.8/
```
This can add support for more devices than is provided by the Julia package manager, however compatibility
is not guaranteed. | SoapySDR | https://github.com/JuliaTelecom/SoapySDR.jl.git |
|
[
"MIT",
"BSL-1.0"
] | 0.5.0 | e96812c38a7ca1c013d35c9515f05a1d023e2191 | docs | 155 | ```@meta
CurrentModule = SoapySDR
```
# SoapySDR High Level API
```@autodocs
Modules = [SoapySDR]
Pages = ["highlevel.jl",
"loghandler.jl"]
```
| SoapySDR | https://github.com/JuliaTelecom/SoapySDR.jl.git |
|
[
"MIT",
"BSL-1.0"
] | 0.5.0 | e96812c38a7ca1c013d35c9515f05a1d023e2191 | docs | 858 | ```@meta
CurrentModule = SoapySDR
```
# SoapySDR
Documentation for [SoapySDR](https://github.com/JuliaTelecom/SoapySDR.jl).
This package provides a Julia wrapper for the [SoapySDR](https://github.com/pothosware/SoapySDR) C++ library.
## Quick Start
### Transmitting and Receiving (loopback)
````@eval
using Markdown
Markdown.parse("""
```julia
$(read(joinpath(@__DIR__, "../../examples/highlevel_loopback.jl"), String))
```
""")
````
## Release Log
### 0.2
This changes the high level API to allow device constructor arguments.
In prior releases to construct a `Device` one would do:
```
devs = Devices()
dev = devs[1]
```
Now one has to explicitly call `open` to create the `Device`, which allows arguments to be set:
```
devs = Devices()
devs[1]["argument"] = "value"
dev = open(devs[1])
```
Similarly it is now possible to `close` a device.
| SoapySDR | https://github.com/JuliaTelecom/SoapySDR.jl.git |
|
[
"MIT",
"BSL-1.0"
] | 0.5.0 | e96812c38a7ca1c013d35c9515f05a1d023e2191 | docs | 400 | ```@meta
CurrentModule = SoapySDR
```
# SoapySDR Low Level API
!!! warning
This documentation is part of the low level libsoapysdr interface.
These bindings and documentation are autogenerated and reflect the
complete SoapySDR C API.
For end-users, the [high-level](./highlevel.md) Julia APIs are preferred.
```@autodocs
Modules = [SoapySDR]
Pages = ["lowlevel/auto_wrap.jl"]
```
| SoapySDR | https://github.com/JuliaTelecom/SoapySDR.jl.git |
|
[
"MIT",
"BSL-1.0"
] | 0.5.0 | e96812c38a7ca1c013d35c9515f05a1d023e2191 | docs | 1487 | # Troubleshooting
Below are some common issues and how to resolve them. If you don't find the issue listed here, please [file an issue](). We are always looking to help identify and fix bugs.
## No Devices Found
You may see:
```
julia> Devices()
No devices available! Make sure a supported SDR module is included.
```
Make sure you have included a module driver from the [module list](./index.md#Loading-a-Driver-Module).
If this doesn't work, and you are on Linux, please see the [udev section](./#Udev-Rules) below.
## Linux Troubleshooting
### Udev Rules
Udev rules should be copied into `/etc/udev/rules.d/`.
Udev rules for some common devices are linked below:
- [RTL-SDR](https://github.com/osmocom/rtl-sdr/blob/d770add42e87a40e59a0185521373f516778384b/rtl-sdr.rules)
- [USRP](https://github.com/EttusResearch/uhd/blob/919043f305efdd29fbdf586e9cde95d9507150e8/host/utils/uhd-usrp.rules)
- [LimeSDR](https://github.com/myriadrf/LimeSuite/blob/a45e482dad28508d8787e0fdc5168d45ac877ab5/udev-rules/64-limesuite.rules)
- [ADALM-Pluto](https://github.com/analogdevicesinc/plutosdr-fw/blob/cbe7306055828ce0a12a9da35efc6685c86f811f/scripts/53-adi-plutosdr-usb.rules)
### Blacklisting Kernel Modules
For some devices such as the RTL-SDR, a kernel module may need to be blacklisted in order to use
the user space driver. Typically the library will warn is this is required. Please check you distribution
instructions for how to do this.
RTL-SDR module name: `dvb_usb_rtl28xxu` | SoapySDR | https://github.com/JuliaTelecom/SoapySDR.jl.git |
|
[
"MIT",
"BSL-1.0"
] | 0.5.0 | e96812c38a7ca1c013d35c9515f05a1d023e2191 | docs | 1793 | # Tutorial
!!! note
You have to load a driver for your particular SDR in order to work
with SoapySDR. Available modules through the Julia Package manager are listed
on the [index.](./index.md)
The entry point of this package is the `Devices()` object, which will list
all devices known to SoapySDR on the current system.
Here we will use XTRX:
```
julia> using SoapySDR, xtrx_jll
julia> Devices()
[1] :addr => "pcie:///dev/xtrx0", :dev => "pcie:///dev/xtrx0", :driver => "xtrx", :label => "XTRX: pcie:///dev/xtrx0 (10Gbit)", :media => "PCIe", :module => "SoapyXTRX", :name => "XTRX", :serial => "", :type => "xtrx"
```
Devices may be selected just by indexing:
```
julia> device = Devices()[1]
SoapySDR xtrxdev device (driver: xtrxsoapy) w/ 2 TX channels and 2 RX channels
```
The channels on the device are then available on the resulting object
```
julia> device.tx
2-element SoapySDR.ChannelList:
Channel(xtrxdev, Tx, 0)
Channel(xtrxdev, Tx, 1)
julia> device.rx
2-element SoapySDR.ChannelList:
Channel(xtrxdev, Rx, 0)
Channel(xtrxdev, Rx, 1)
julia> device.tx[1]
TX Channel #1 on xtrxdev
Selected Antenna [TXH, TXW]: TXW
Bandwidth [ 800 kHz .. 16 MHz, 28..60 MHz ]: 0.0 Hz
Frequency [ 30 MHz .. 3.8 GHz ]: 0.0 Hz
RF [ 30 MHz .. 3.8 GHz ]: 0.0 Hz
BB [ -00..00 Hz ]: 0.0 Hz
Gain [0.0 dB .. 52.0 dB]: 0.0 dB
PAD [0.0 dB .. 52.0 dB]: 0.0 dB
Sample Rate [ 2.1..56.2 MHz, 61.4..80 MHz ]: 0.0 Hz
DC offset correction: (0.0, 0.0)
IQ balance correction: (0.0, 0.0)
```
To send or receive data, start a stream on a particular channel:
```
julia> stream = SDRStream(ComplexF32, [device.rx[1]])
Stream on xtrxdev
```
These may then be accessed using standard IO functions:
```
julia> SoapySDR.activate!(stream)
julia> Base.read(stream, 10_000)
```
| SoapySDR | https://github.com/JuliaTelecom/SoapySDR.jl.git |
|
[
"MIT",
"BSL-1.0"
] | 0.5.0 | e96812c38a7ca1c013d35c9515f05a1d023e2191 | docs | 240 | # SoapySDR Binding generation via Clang.jl
Instructions:
```
] activate .
] instantiate
] up
include("gen.jl")
```
This generates the files in `src/lowlevel/<header>.h.jl`. Files in lowlevel without this extension are maintained by hand. | SoapySDR | https://github.com/JuliaTelecom/SoapySDR.jl.git |
|
[
"MIT"
] | 1.6.0 | a7dc7bfc2fc6638c18241bfcdb9d73c7dd256482 | code | 5037 | # override Documenter.jl/src/Writers/HTMLWriter.jl
using Dates
import Documenter: Documents, Documenter, Writers, Utilities
import Documenter.Utilities.DOM: DOM, Tag, @tags
import Documenter.Writers.HTMLWriter:
getpage,
render_head,
render_article,
open_output,
mdconvert,
pagetitle,
navhref,
domify,
render_settings,
THEMES
const zh_CN_numbers = ["一", "二", "三", "四", "五", "六",
"七", "八", "九", "十", "十一", "十二"]
const zh_CN_months = zh_CN_numbers[1:12] .* "月"
const zh_CN_months_abbrev = zh_CN_months
const zh_CN_days = [
"周" .* zh_CN_numbers[1:6]
"周日"
]
Dates.LOCALES["zh_CN"] = Dates.DateLocale(zh_CN_months, zh_CN_months_abbrev, zh_CN_days, [""])
#=
1. 修改编辑翻译提示语
- 改 icon 为 FontAwesome: fa-globe(f0ac)
- 改 "Edit on Github" 为 “完善 Transifex 上的翻译"
- 改为对应的 Transifex URL
2. 修改前后翻页提示语
- "Previous" => t_Previous
- "Next" => t_Next
=#
const t_Edit_on_xx = " 完善 Transifex 上的翻译" # 开头加空格,避免与 icon 靠太近
const t_Icon = "\uf0ac" # fa-globe
# 生成对应文件在 Transifex 上的翻译地址
function transifex_url(rel_path)
# 首页源文件在 GitHub 上
if splitdir(rel_path) == splitdir("src/index.md")
return "https://github.com/JuliaCN/JuliaZH.jl/blob/master/doc/src/index.md"
end
# rel_path => "src\\manual\\getting-started.md"
paths, file_name = splitdir(rel_path)
# paths => "src\\manual"
# file_name => "getting-started.md"
_, parent_fold = splitdir(paths)
# parent_fold => "manual"
page_name = replace(file_name, '.' => "")
# page_name => "getting-startedmd"
# final URL =>
# "https://www.transifex.com/juliacn/manual-zh_cn/translate/#zh_CN/getting-startedmd"
"https://www.transifex.com/juliacn/$parent_fold-zh_cn/translate/#zh_CN/$page_name"
end
# workaround on Documenter/#977
function Documenter.Writers.HTMLWriter.render_navbar(ctx, navnode, edit_page_link::Bool)
@tags div header nav ul li a span
# The breadcrumb (navigation links on top)
navpath = Documents.navpath(navnode)
header_links = map(navpath) do nn
title = mdconvert(pagetitle(ctx, nn); droplinks=true)
nn.page === nothing ? li(a[".is-disabled"](title)) : li(a[:href => navhref(ctx, nn, navnode)](title))
end
header_links[end] = header_links[end][".is-active"]
breadcrumb = nav[".breadcrumb"](
ul[".is-hidden-mobile"](header_links),
ul[".is-hidden-tablet"](header_links[end]) # when on mobile, we only show the page title, basically
)
# The "Edit on GitHub" links and the hamburger to open the sidebar (on mobile) float right
navbar_right = div[".docs-right"]
# Set the logo and name for the "Edit on.." button.
if edit_page_link && (ctx.settings.edit_link !== nothing) && !ctx.settings.disable_git
host_type = Utilities.repo_host_from_url(ctx.doc.user.repo)
logo = t_Icon
pageurl = get(getpage(ctx, navnode).globals.meta, :EditURL, getpage(ctx, navnode).source)
edit_branch = isa(ctx.settings.edit_link, String) ? ctx.settings.edit_link : nothing
# 1.3: 改 URL
url = transifex_url(getpage(ctx, navnode).source)
if url !== nothing
title = t_Edit_on_xx
push!(navbar_right.nodes,
a[".docs-edit-link", :href => url, :title => title](
span[".docs-icon.fab"](logo),
span[".docs-label.is-hidden-touch"](title)
)
)
end
end
# Settings cog
push!(navbar_right.nodes, a[
"#documenter-settings-button.docs-settings-button.fas.fa-cog",
:href => "#", :title => "设置",
])
# Hamburger on mobile
push!(navbar_right.nodes, a[
"#documenter-sidebar-button.docs-sidebar-button.fa.fa-bars.is-hidden-desktop",
:href => "#"
])
# Construct the main <header> node that should be the first element in div.docs-main
header[".docs-navbar"](breadcrumb, navbar_right)
end
function render_settings(ctx)
@tags div header section footer p button hr span select option label a
theme_selector = p(
label[".label"]("选择主题"),
div[".select"](
select["#documenter-themepicker"](option[:value=>theme](theme) for theme in THEMES)
)
)
now_full = Dates.format(now(), "Y U d E HH:MM"; locale="zh_CN")
now_short = Dates.format(now(), "Y U d E"; locale="zh_CN")
buildinfo = p(
"本文档在",
span[".colophon-date", :title => now_full](now_short),
"由",
a[:href => "https://github.com/JuliaDocs/Documenter.jl"]("Documenter.jl"),
"使用$(Base.VERSION)版本的Julia生成。"
)
div["#documenter-settings.modal"](
div[".modal-background"],
div[".modal-card"](
header[".modal-card-head"](
p[".modal-card-title"]("设置"),
button[".delete"]()
),
section[".modal-card-body"](
theme_selector, hr(), buildinfo
),
footer[".modal-card-foot"]()
)
)
end
| JuliaZH | https://github.com/JuliaCN/JuliaZH.jl.git |
|
[
"MIT"
] | 1.6.0 | a7dc7bfc2fc6638c18241bfcdb9d73c7dd256482 | code | 2657 | using DocumenterLaTeX, Markdown
import Documenter: Documents, Documenter, Writers, Utilities
import Documenter.Writers.LaTeXWriter: piperun, _print
const LaTeX_CC="xelatex"
const DOCKER_IMAGE = "tianjun2018/documenter-latex:latest"
function Documenter.Writers.LaTeXWriter.latexinline(io, math::Markdown.LaTeX)
# Handle MathJax and TeX inconsistency since the first wants `\LaTeX` wrapped
# in math delims, whereas actual TeX fails when that is done.
math.formula == "\\LaTeX" ? _print(io, " ", math.formula, " ") : _print(io, " \\(", math.formula, "\\) ")
end
function Documenter.Writers.LaTeXWriter.compile_tex(doc::Documents.Document, settings::LaTeX, texfile::String)
if settings.platform == "native"
Sys.which("latexmk") === nothing && (@error "LaTeXWriter: latexmk command not found."; return false)
@info "LaTeXWriter: using latexmk to compile tex."
piperun(`latexmk -f -interaction=nonstopmode -view=none -$(LaTeX_CC) -shell-escape $texfile`)
return true
# NOTE: 中文排版依然有问题,我们暂时不管他们。输出的pdf大部分情况下依然可以使用。
# try
# piperun(`latexmk -f -interaction=nonstopmode -view=none -$(LaTeX_CC) -shell-escape $texfile`)
# return true
# catch err
# logs = cp(pwd(), mktempdir(); force=true)
# @error "LaTeXWriter: failed to compile tex with latexmk. " *
# "Logs and partial output can be found in $(Utilities.locrepr(logs))." exception = err
# return false
# end
elseif settings.platform == "docker"
Sys.which("docker") === nothing && (@error "LaTeXWriter: docker command not found."; return false)
@info "LaTeXWriter: using docker to compile tex."
script = """
mkdir /home/zeptodoctor/build
cd /home/zeptodoctor/build
cp -r /mnt/. .
latexmk -f -interaction=nonstopmode -view=none -$(LaTeX_CC) -shell-escape $texfile
"""
try
piperun(`docker run -itd -u zeptodoctor --name latex-container -v $(pwd()):/mnt/ --rm $(DOCKER_IMAGE)`)
piperun(`docker exec -u zeptodoctor latex-container bash -c $(script)`)
piperun(`docker cp latex-container:/home/zeptodoctor/build/. .`)
return true
catch err
logs = cp(pwd(), mktempdir(); force=true)
@error "LaTeXWriter: failed to compile tex with docker. " *
"Logs and partial output can be found in $(Utilities.locrepr(logs))." exception = err
return false
finally
try; piperun(`docker stop latex-container`); catch; end
end
end
end
| JuliaZH | https://github.com/JuliaCN/JuliaZH.jl.git |
|
[
"MIT"
] | 1.6.0 | a7dc7bfc2fc6638c18241bfcdb9d73c7dd256482 | code | 9190 | #!/usr/bin/env julia
# This file is a part of Julia. License is MIT: https://julialang.org/license
using Libdl
# Build a system image binary at sysimg_path.dlext. Allow insertion of a userimg via
# userimg_path. If sysimg_path.dlext is currently loaded into memory, don't continue
# unless force is set to true. Allow targeting of a CPU architecture via cpu_target.
function default_sysimg_path(debug=false)
if Sys.isunix()
splitext(Libdl.dlpath(debug ? "sys-debug" : "sys"))[1]
else
joinpath(dirname(Sys.BINDIR), "lib", "julia", debug ? "sys-debug" : "sys")
end
end
"""
build_sysimg(sysimg_path=default_sysimg_path(), cpu_target="native", userimg_path=nothing; force=false)
Rebuild the system image. Store it in `sysimg_path`, which defaults to a file named `sys.ji`
that sits in the same folder as `libjulia.{so,dylib}`, except on Windows where it defaults
to `Sys.BINDIR/../lib/julia/sys.ji`. Use the cpu instruction set given by `cpu_target`.
Valid CPU targets are the same as for the `-C` option to `julia`, or the `-march` option to
`gcc`. Defaults to `native`, which means to use all CPU instructions available on the
current processor. Include the user image file given by `userimg_path`, which should contain
directives such as `using MyPackage` to include that package in the new system image. New
system image will not replace an older image unless `force` is set to true.
"""
function build_sysimg(sysimg_path=nothing, cpu_target="native", userimg_path=nothing; force=false, debug=false)
if sysimg_path === nothing
sysimg_path = default_sysimg_path(debug)
end
# Quit out if a sysimg is already loaded and is in the same spot as sysimg_path, unless forcing
sysimg = Libdl.dlopen_e("sys")
if sysimg != C_NULL
if !force && Base.samefile(Libdl.dlpath(sysimg), "$(sysimg_path).$(Libdl.dlext)")
@info("System image already loaded at $(Libdl.dlpath(sysimg)), set force=true to override.")
return nothing
end
end
# Canonicalize userimg_path before we enter the base_dir
if userimg_path !== nothing
userimg_path = abspath(userimg_path)
end
# Enter base and setup some useful paths
base_dir = dirname(Base.find_source_file("sysimg.jl"))
cd(base_dir) do
julia = joinpath(Sys.BINDIR, debug ? "julia-debug" : "julia")
cc, warn_msg = find_system_compiler()
# Ensure we have write-permissions to wherever we're trying to write to
try
touch("$sysimg_path.ji")
catch
err_msg = "Unable to modify $sysimg_path.ji, ensure parent directory exists "
err_msg *= "and is writable; absolute paths work best.)"
error(err_msg)
end
# Copy in userimg.jl if it exists
if userimg_path !== nothing
if !isfile(userimg_path)
error("$userimg_path is not found, ensure it is an absolute path.")
end
if isfile("userimg.jl")
error("$(joinpath(base_dir, "userimg.jl")) already exists, delete manually to continue.")
end
cp(userimg_path, "userimg.jl")
end
try
# Start by building basecompiler.{ji,o}
basecompiler_path = joinpath(dirname(sysimg_path), "basecompiler")
@info("Building basecompiler.o")
@info("$julia -C $cpu_target --output-ji $basecompiler_path.ji --output-o $basecompiler_path.o compiler/compiler.jl")
run(`$julia -C $cpu_target --output-ji $basecompiler_path.ji --output-o $basecompiler_path.o compiler/compiler.jl`)
# Bootstrap off of that to create sys.{ji,o}
@info("Building sys.o")
@info("$julia -C $cpu_target --output-ji $sysimg_path.ji --output-o $sysimg_path.o -J $basecompiler_path.ji --startup-file=no sysimg.jl")
run(`$julia -C $cpu_target --output-ji $sysimg_path.ji --output-o $sysimg_path.o -J $basecompiler_path.ji --startup-file=no sysimg.jl`)
if cc !== nothing
link_sysimg(sysimg_path, cc, debug)
end
# Spit out all our warning messages
for w in warn_msg
@warn w
end
if cc == nothing
@info("System image successfully built at $sysimg_path.ji.")
end
if !Base.samefile("$(default_sysimg_path(debug)).ji", "$sysimg_path.ji")
if isfile("$sysimg_path.$(Libdl.dlext)")
@info("To run Julia with this image loaded, run: `julia -J $sysimg_path.$(Libdl.dlext)`.")
else
@info("To run Julia with this image loaded, run: `julia -J $sysimg_path.ji`.")
end
else
@info("Julia will automatically load this system image at next startup.")
end
finally
# Cleanup userimg.jl
if userimg_path !== nothing && isfile("userimg.jl")
rm("userimg.jl")
end
end
end
end
# Search for a compiler to link sys.o into sys.dl_ext. Honor CC environment variable.
function find_system_compiler()
warn_msg = String[] # save warning messages into an array
if haskey(ENV, "CC")
if !success(`$(ENV["CC"]) -v`)
push!(warn_msg, "Using compiler override $(ENV["CC"]), but unable to run `$(ENV["CC"]) -v`.")
end
return ENV["CC"], warn_msg
end
# On Windows, check to see if WinRPM is installed, and if so, see if gcc is installed
if Sys.iswindows()
try
Core.eval(Main, :(using WinRPM))
winrpmgcc = joinpath(WinRPM.installdir, "usr", "$(Sys.ARCH)-w64-mingw32",
"sys-root", "mingw", "bin", "gcc.exe")
if success(`$winrpmgcc --version`)
return winrpmgcc, warn_msg
else
throw()
end
catch
push!(warn_msg, "Install GCC via `Pkg.add(\"WinRPM\"); WinRPM.install(\"gcc\")` to generate sys.dll for faster startup times.")
end
end
# See if `cc` exists
try
if success(`cc -v`)
return "cc", warn_msg
end
catch
push!(warn_msg, "No supported compiler found; startup times will be longer.")
return nothing, warn_msg
end
end
# Link sys.o into sys.$(dlext)
function link_sysimg(sysimg_path=nothing, cc=find_system_compiler(), debug=false)
if sysimg_path === nothing
sysimg_path = default_sysimg_path(debug)
end
julia_libdir = dirname(Libdl.dlpath(debug ? "libjulia-debug" : "libjulia"))
FLAGS = ["-L$julia_libdir"]
push!(FLAGS, "-shared")
push!(FLAGS, debug ? "-ljulia-debug" : "-ljulia")
if Sys.iswindows()
push!(FLAGS, "-lssp")
end
if Sys.isapple()
whole_archive = "-Wl,-all_load"
no_whole_archive = ""
else
whole_archive = "-Wl,--whole-archive"
no_whole_archive = "-Wl,--no-whole-archive"
end
sysimg_file = "$sysimg_path.$(Libdl.dlext)"
cc_cmd = `$cc $FLAGS -o $sysimg_path.tmp $whole_archive $sysimg_path.o $no_whole_archive`
@info("Linking sys.$(Libdl.dlext) with $cc_cmd")
# Windows has difficulties overwriting a file in use so we first link to a temp file
if success(pipeline(cc_cmd; stdout=stdout, stderr=stderr))
mv("$sysimg_path.tmp", sysimg_file; force=true)
end
@info("System image successfully built at $sysimg_path.$(Libdl.dlext)")
return
end
# When running this file as a script, try to do so with default values. If arguments are passed
# in, use them as the arguments to build_sysimg above.
# Also check whether we are running `genstdlib.jl`, in which case we don't want to build a
# system image and instead only need `build_sysimg`'s docstring to be available.
if !isdefined(Main, :GenStdLib) && !isinteractive()
if length(ARGS) > 5 || ("--help" in ARGS || "-h" in ARGS)
println("Usage: build_sysimg.jl <sysimg_path> <cpu_target> <usrimg_path.jl> [--force] [--debug] [--help]")
println(" <sysimg_path> is an absolute, extensionless path to store the system image at")
println(" <cpu_target> is an LLVM cpu target to build the system image against")
println(" <usrimg_path.jl> is the path to a user image to be baked into the system image")
println(" --debug Using julia-debug instead of julia to build the system image")
println(" --force Set if you wish to overwrite the default system image")
println(" --help Print out this help text and exit")
println()
println(" Example:")
println(" build_sysimg.jl /usr/local/lib/julia/sys core2 ~/my_usrimg.jl --force")
println()
println(" Running this script with no arguments is equivalent to:")
println(" build_sysimg.jl $(default_sysimg_path()) native")
return 0
end
debug_flag = "--debug" in ARGS
filter!(x -> x != "--debug", ARGS)
force_flag = "--force" in ARGS
filter!(x -> x != "--force", ARGS)
build_sysimg(ARGS...; force=force_flag, debug=debug_flag)
end
| JuliaZH | https://github.com/JuliaCN/JuliaZH.jl.git |
|
[
"MIT"
] | 1.6.0 | a7dc7bfc2fc6638c18241bfcdb9d73c7dd256482 | code | 7055 | # tweaked from https://github.com/JuliaLang/julia/blob/master/doc/make.jl
# Install dependencies needed to build the documentation.
empty!(LOAD_PATH)
push!(LOAD_PATH, @__DIR__, "@stdlib")
empty!(DEPOT_PATH)
pushfirst!(DEPOT_PATH, joinpath(@__DIR__, "deps"))
using Pkg
Pkg.instantiate()
using Documenter, DocumenterLaTeX
include("../contrib/HTMLWriter.jl")
include("../contrib/LaTeXWriter.jl")
# Include the `build_sysimg` file.
baremodule GenStdLib end
@isdefined(build_sysimg) || @eval module BuildSysImg
include(joinpath(@__DIR__, "..", "contrib", "build_sysimg.jl"))
end
# Documenter Setup.
symlink_q(tgt, link) = isfile(link) || symlink(tgt, link)
cp_q(src, dest) = isfile(dest) || cp(src, dest)
"""
cpi18ndoc(;root, i18ndoc, stdlib)
Copy i18n doc to build folder.
"""
function cpi18ndoc(;
root=joinpath(@__DIR__, ".."),
i18ndoc=["base", "devdocs", "manual"], stdlib=true, force=false)
# stdlib
if stdlib
cp(joinpath(root, "zh_CN", "stdlib"), joinpath(root, "stdlib"); force=force)
end
for each in i18ndoc
cp(joinpath(root, "zh_CN", "doc", "src", each), joinpath(root, "doc", "src", each); force=force)
end
end
"""
clean(;root, stdlib, i18ndoc)
Clean up build i18n cache.
"""
function clean(;root=joinpath(@__DIR__, ".."), stdlib=true, i18ndoc=["base", "devdocs", "manual"])
if stdlib
rm(joinpath(root, "stdlib"); recursive=true)
end
for each in i18ndoc
rm(joinpath(root, "doc", "src", each); recursive=true)
end
end
cpi18ndoc(;force=true)
# make links for stdlib package docs, this is needed until #522 in Documenter.jl is finished
const STDLIB_DOCS = []
const STDLIB_DIR = joinpath(@__DIR__, "..", "stdlib")
cd(joinpath(@__DIR__, "src")) do
Base.rm("stdlib"; recursive=true, force=true)
mkdir("stdlib")
for dir in readdir(STDLIB_DIR)
sourcefile = joinpath(STDLIB_DIR, dir, "docs", "src", "index.md")
if isfile(sourcefile)
targetfile = joinpath("stdlib", dir * ".md")
push!(STDLIB_DOCS, (stdlib = Symbol(dir), targetfile = targetfile))
if Sys.iswindows()
cp_q(sourcefile, targetfile)
else
symlink_q(sourcefile, targetfile)
end
end
end
end
# manual/unicode-input.md
const UnicodeDataPath = joinpath(Sys.BINDIR, "..", "UnicodeData.txt")
if !isfile(UnicodeDataPath)
download("http://www.unicode.org/Public/9.0.0/ucd/UnicodeData.txt", UnicodeDataPath)
end
const PAGES = [
"主页" => "index.md",
"手册" => [
"manual/getting-started.md",
"manual/variables.md",
"manual/integers-and-floating-point-numbers.md",
"manual/mathematical-operations.md",
"manual/complex-and-rational-numbers.md",
"manual/strings.md",
"manual/functions.md",
"manual/control-flow.md",
"manual/variables-and-scoping.md",
"manual/types.md",
"manual/methods.md",
"manual/constructors.md",
"manual/conversion-and-promotion.md",
"manual/interfaces.md",
"manual/modules.md",
"manual/documentation.md",
"manual/metaprogramming.md",
"manual/arrays.md",
"manual/missing.md",
"manual/networking-and-streams.md",
"manual/parallel-computing.md",
"manual/asynchronous-programming.md",
"manual/multi-threading.md",
"manual/distributed-computing.md",
"manual/running-external-programs.md",
"manual/calling-c-and-fortran-code.md",
"manual/handling-operating-system-variation.md",
"manual/environment-variables.md",
"manual/embedding.md",
"manual/code-loading.md",
"manual/profile.md",
"manual/stacktraces.md",
"manual/performance-tips.md",
"manual/workflow-tips.md",
"manual/style-guide.md",
"manual/faq.md",
"manual/noteworthy-differences.md",
"manual/unicode-input.md",
"manual/command-line-options.md",
],
"Base" => [
"base/base.md",
"base/collections.md",
"base/math.md",
"base/numbers.md",
"base/strings.md",
"base/arrays.md",
"base/parallel.md",
"base/multi-threading.md",
"base/constants.md",
"base/file.md",
"base/io-network.md",
"base/punctuation.md",
"base/sort.md",
"base/iterators.md",
"base/c.md",
"base/libc.md",
"base/stacktraces.md",
"base/simd-types.md",
],
"Standard Library" =>
[stdlib.targetfile for stdlib in STDLIB_DOCS],
"Developer Documentation" => [
"devdocs/reflection.md",
"Documentation of Julia's Internals" => [
"devdocs/init.md",
"devdocs/ast.md",
"devdocs/types.md",
"devdocs/object.md",
"devdocs/eval.md",
"devdocs/callconv.md",
"devdocs/compiler.md",
"devdocs/functions.md",
"devdocs/cartesian.md",
"devdocs/meta.md",
"devdocs/subarrays.md",
"devdocs/isbitsunionarrays.md",
"devdocs/sysimg.md",
"devdocs/llvm.md",
"devdocs/stdio.md",
"devdocs/boundscheck.md",
"devdocs/locks.md",
"devdocs/offset-arrays.md",
"devdocs/require.md",
"devdocs/inference.md",
"devdocs/ssair.md",
"devdocs/gc-sa.md",
],
"Developing/debugging Julia's C code" => [
"devdocs/backtraces.md",
"devdocs/debuggingtips.md",
"devdocs/valgrind.md",
"devdocs/sanitizers.md",
# "devdocs/probes.md" # seems not synced from transifix
],
],
]
for stdlib in STDLIB_DOCS
@eval using $(stdlib.stdlib)
end
const render_pdf = "pdf" in ARGS
const is_deploy = "deploy" in ARGS
const format = if render_pdf
LaTeX(
platform = "texplatform=docker" in ARGS ? "docker" : "native"
)
else
Documenter.HTML(
prettyurls = is_deploy,
canonical = is_deploy ? "https://juliacn.github.io/JuliaZH.jl/latest/" : nothing,
analytics = "UA-28835595-9",
assets = [
"assets/julia-manual.css"
],
lang = "zh-cn",
# footer = "📢📢📢Julia中文社区现已加入“开源软件供应链点亮计划”,如果你想改善Julia中文文档的翻译,那就赶快来 [报名](https://summer.iscas.ac.cn/#/org/prodetail/210370191) 吧!"
)
end
makedocs(
modules = [Base, Core, BuildSysImg, [Base.root_module(Base, stdlib.stdlib) for stdlib in STDLIB_DOCS]...],
clean = true,
doctest = ("doctest=fix" in ARGS) ? (:fix) : ("doctest=true" in ARGS) ? true : false,
linkcheck = "linkcheck=true" in ARGS,
checkdocs = :none,
format = format,
sitename = "Julia中文文档",
authors = "Julia中文社区",
pages = PAGES,
)
deploydocs(
repo = "github.com/JuliaCN/JuliaZH.jl.git",
target = "build",
deps = nothing,
make = nothing,
branch = render_pdf ? "pdf" : "gh-pages"
)
| JuliaZH | https://github.com/JuliaCN/JuliaZH.jl.git |
|
[
"MIT"
] | 1.6.0 | a7dc7bfc2fc6638c18241bfcdb9d73c7dd256482 | code | 536 | module JuliaZH
import Base.Docs: DocStr
# early version of JuliaZH provides these two methods
import PkgServerClient: set_mirror, generate_startup
const keywords = Dict{Symbol,DocStr}()
function __init__()
# Set pkg server to the nearest mirror
@eval using PkgServerClient
# set to Chinese env by default
ENV["REPL_LOCALE"] = "zh_CN"
if ccall(:jl_generating_output, Cint, ()) == 0
include(joinpath(@__DIR__, "i18n_patch.jl"))
include(joinpath(@__DIR__, "basedocs.jl"))
end
end
end # module
| JuliaZH | https://github.com/JuliaCN/JuliaZH.jl.git |
|
[
"MIT"
] | 1.6.0 | a7dc7bfc2fc6638c18241bfcdb9d73c7dd256482 | code | 11505 | import Base.BaseDocs: @kw_str
"""
**欢迎来到 Julia $(string(VERSION)).** 完整的中文手册可以在这里找到
https://docs.juliacn.com/
更多中文资料和教程,也请关注 Julia 中文社区
https://cn.julialang.org
新手请参考中文 discourse 上的新手指引
https://discourse.juliacn.com/t/topic/159
输入 `?`, 然后输入你想要查看帮助文档的函数或者宏名称就可以查看它们的文档。例如 `?cos`, 或者 `?@time` 然后按回车键即可。
在 REPL 中输入 `ENV["REPL_LOCALE"]=""` 将恢复英文模式。再次回到中文模式请输入 `ENV["REPL_LOCALE"]="zh_CN"`。
"""
kw"help", kw"?", kw"julia", kw""
"""
using
`using Foo` 将会加载一个名为 `Foo` 的模块(module)或者一个包,然后其 [`export`](@ref) 的名称将可以直接使用。不论是否被 `export`,名称都可以通过点来访问(例如,输入 `Foo.foo` 来访问到 `foo`)。查看[手册中关于模块的部分](@ref modules)以获取更多细节。
"""
kw"using"
"""
import
`import Foo` 将会加载一个名为 `Foo` 的模块(module)或者一个包。`Foo` 模块中的名称可以通过点来访问到(例如,输入 `Foo.foo` 可以获取到 `foo`)。查看[手册中关于模块的部分](@ref modules)以获取更多细节。
"""
kw"import"
"""
export
`export` 被用来在模块中告诉Julia哪些函数或者名字可以由用户使用。例如 `export foo` 将在 [`using`](@ref) 这个 module 的时候使得 `foo`可以直接被访问到。查看[手册中关于模块的部分](@ref modules)以获取更多细节。
"""
kw"export"
"""
abstract type
`abstract type` 声明来一个不能实例化的类型,它将仅仅作为类型图中的一个节点存在,从而能够描述一系列相互关联的具体类型(concrete type):这些具体类型都是抽象类型的子节点。抽象类型在概念上使得 Julia 的类型系统不仅仅是一系列对象的集合。例如:
```julia
abstract type Number end
abstract type Real <: Number end
```
[`Number`](@ref) 没有父节点(父类型), 而 [`Real`](@ref) 是 `Number` 的一个抽象子类型。
"""
kw"abstract type"
"""
module
`module` 会声明一个 `Module` 类型的实例用于描述一个独立的变量名空间。在一个模块(module)里,你可以控制来自于其它模块的名字是否可见(通过载入,import),你也可以决定你的名字有哪些是可以公开的(通过暴露,export)。模块使得你在在创建上层定义时无需担心命名冲突。查看[手册中关于模块的部分](@ref modules)以获取更多细节。
# 例子
```julia
module Foo
import Base.show
export MyType, foo
struct MyType
x
end
bar(x) = 2x
foo(a::MyType) = bar(a.x) + 1
show(io::IO, a::MyType) = print(io, "MyType \$(a.x)")
end
```
"""
kw"module"
"""
baremodule
`baremodule` 将声明一个不包含 `using Base` 或者 `eval` 定义的模块。但是它将仍然载入 `Core` 模块。
"""
kw"baremodule"
"""
primitive type
`primitive type` 声明了一个其数据仅仅由一系列二进制数表示的具体类型。比较常见的例子是整数类型和浮点类型。下面是一些内置的原始类型(primitive type):
```julia
primitive type Char 32 end
primitive type Bool <: Integer 8 end
```
名称后面的数字表达了这个类型存储所需的比特数目。目前这个数字要求是 8 bit 的倍数。[`Bool`](@ref) 类型的声明展示了一个原始类型如何选择成为另一个类型的子类型。
"""
kw"primitive type"
"""
macro
`macro` 定义了一种会将生成的代码包含在最终程序体中的方法,这称之为宏。一个宏将一系列输入映射到一个表达式,然后所返回的表达式将会被直接进行编译而不需要在运行时调用 `eval` 函数。宏的输入可以包括表达式、字面量和符号。例如:
# 例子
```jldoctest
julia> macro sayhello(name)
return :( println("Hello, ", \$name, "!") )
end
@sayhello (macro with 1 method)
julia> @sayhello "小明"
Hello, 小明!
```
"""
kw"macro"
"""
local
`local`将会定义一个新的局部变量。
查看[手册:变量作用域](@ref scope-of-variables)以获取更详细的信息。
# 例子
```jldoctest
julia> function foo(n)
x = 0
for i = 1:n
local x # introduce a loop-local x
x = i
end
x
end
foo (generic function with 1 method)
julia> foo(10)
0
```
"""
kw"local"
"""
global
`global x` 将会使得当前作用域和当前作用所包含的作用域里的 `x` 指向名为 `x` 的全局变量。查看[手册:变量作用域](@ref scope-of-variables)以获取更多信息。
# 例子
```jldoctest
julia> z = 3
3
julia> function foo()
global z = 6 # use the z variable defined outside foo
end
foo (generic function with 1 method)
julia> foo()
6
julia> z
6
```
"""
kw"global"
"""
let
`let` 会在每次被运行时声明一个新的变量绑定。这个新的变量绑定将拥有一个新的地址。这里的不同只有当变量通过闭包生存在它们的作用域外时才会显现。`let` 语法接受逗号分割的一系列赋值语句和变量名:
```julia
let var1 = value1, var2, var3 = value3
code
end
```
这些赋值语句是按照顺序求值的,等号右边的表达式将会首先求值,然后才绑定给左边的变量。因此这使得 `let x = x` 这样的表达式有意义,因为这两个 `x` 变量将具有不同的地址。
"""
kw"let"
"""
quote
`quote` 会将其包含的代码扩变成一个多重的表达式对象,而无需显示调用 `Expr` 的构造器。这称之为引用,比如说
```julia
ex = quote
x = 1
y = 2
x + y
end
```
和其它引用方式不同的是,`:( ... )`形式的引用(被包含时)将会在表达式树里引入一个在操作表达式树时必须要考虑的 `QuoteNode` 元素。而在其它场景下,`:( ... )`和 `quote .. end` 代码块是被同等对待的。
"""
kw"quote"
"""
'
厄米算符(共轭转置),参见 [`adjoint`](@ref)
# 例子
```jldoctest
julia> A = [1.0 -2.0im; 4.0im 2.0]
2×2 Array{Complex{Float64},2}:
1.0+0.0im -0.0-2.0im
0.0+4.0im 2.0+0.0im
julia> A'
2×2 Array{Complex{Float64},2}:
1.0-0.0im 0.0-4.0im
-0.0+2.0im 2.0-0.0im
```
"""
kw"'"
"""
const
`const` 被用来声明常数全局变量。在大部分(尤其是性能敏感的代码)全局变量应当被声明为常数。
```julia
const x = 5
```
可以使用单个 `const` 声明多个常数变量。
```julia
const y, z = 7, 11
```
注意 `const` 只会作用于一个 `=` 操作,因此 `const x = y = 1` 声明了 `x` 是常数,而 `y` 不是。在另一方面,`const x = const y = 1`声明了 `x` 和 `y` 都是常数。
注意「常数性质」并不会强制容器内部变成常数,所以如果 `x` 是一个数组或者字典(举例来讲)你仍然可以给它们添加或者删除元素。
严格来讲,你甚至可以重新定义 `const`(常数)变量,尽管这将会让编译器产生一个警告。唯一严格的要求是这个变量的**类型**不能改变,这也是为什么常数变量会比一般的全局变量更快的原因。
"""
kw"const"
"""
function
函数由 `function` 关键词定义:
```julia
function add(a, b)
return a + b
end
```
或者是更短的形式:
```julia
add(a, b) = a + b
```
[`return`](@ref) 关键词的使用方法和其它语言完全一样,但是常常是不使用的。一个没有显示声明 `return` 的函数将返回函数体最后一个表达式。
"""
kw"function"
"""
return
`return` 可以用来在函数体中立即退出并返回给定值,例如
```julia
function compare(a, b)
a == b && return "equal to"
a < b ? "less than" : "greater than"
end
```
通常,你可以在函数体的任意位置放置 `return` 语句,包括在多层嵌套的循环和条件表达式中,但要注意 `do` 块。例如:
```julia
function test1(xs)
for x in xs
iseven(x) && return 2x
end
end
function test2(xs)
map(xs) do x
iseven(x) && return 2x
x
end
end
```
在第一个例子中,return 一碰到偶数就跳出包含它的函数,因此 `test1([5,6,7])` 返回 `12`。
你可能希望第二个例子的行为与此相同,但实际上,这里的 `return` 只会跳出(在 `do` 块中的)*内部*函数并把值返回给 `map`。于是,`test2([5,6,7])` 返回 `[5,12,7]`。
"""
kw"return"
# 仿照 https://docs.juliacn.com/latest/manual/control-flow/#man-conditional-evaluation-1
"""
if/elseif/else
`if`/`elseif`/`else` 执行条件表达式(Conditional evaluation)可以根据布尔表达式的值,让部分代码被执行或者不被执行。下面是对 `if`-`elseif`-`else` 条件语法的分析:
```julia
if x < y
println("x is less than y")
elseif x > y
println("x is greater than y")
else
println("x is equal to y")
end
```
如果表达式 `x < y` 是 `true`,那么对应的代码块会被执行;否则判断条件表达式 `x > y`,如果它是 `true`,则执行对应的代码块;如果没有表达式是 true,则执行 `else` 代码块。`elseif` 和 `else` 代码块是可选的,并且可以使用任意多个 `elseif` 代码块。
"""
kw"if", kw"elseif", kw"else"
"""
for
`for` 循环通过迭代一系列值来重复计算循环体。
# 例子
```jldoctest
julia> for i in [1, 4, 0]
println(i)
end
1
4
0
```
"""
kw"for"
# 仿照 https://docs.juliacn.com/latest/manual/control-flow/#man-loops-1
"""
while
`while` 循环会重复执行条件表达式,并在该表达式为 `true` 时继续执行 while 循环的主体部分。当 while 循环第一次执行时,如果条件表达式为 false,那么主体代码就一次也不会被执行。
# 例子
jldoctest
julia> i = 1
1
julia> while i < 5
println(i)
global i += 1
end
1
2
3
4
```
"""
kw"while"
"""
end
`end` 标记一个表达式块的结束,例如 [`module`](@ref)、[`struct`](@ref)、[`mutable struct`](@ref)、[`begin`](@ref)、[`let`](@ref)、[`for`](@ref) 等。`end` 在索引数组时也可以用来表示维度的最后一个索引。
# 例子
```jldoctest
julia> A = [1 2; 3 4]
2×2 Array{Int64,2}:
1 2
3 4
julia> A[end, :]
2-element Array{Int64,1}:
3
4
```
"""
kw"end"
# 仿照 https://docs.juliacn.com/latest/manual/control-flow/#try/catch-%E8%AF%AD%E5%8F%A5-1
"""
try/catch
`try/catch` 语句可以用来捕获 `Exception`,并进行异常处理。例如,一个自定义的平方根函数可以通过 `Exception` 来实现自动按需调用求解实数或者复数平方根的方法:
```julia
f(x) = try
sqrt(x)
catch
sqrt(complex(x, 0))
end
```
`try/catch` 语句允许保存 `Exception` 到一个变量中,例如 `catch y`。
`try/catch` 组件的强大之处在于能够将高度嵌套的计算立刻解耦成更高层次地调用函数。
"""
kw"try", kw"catch"
# 仿照 https://docs.juliacn.com/latest/manual/control-flow/#finally-%E5%AD%90%E5%8F%A5-1
"""
finally
无论代码块是如何退出的,都让代码块在退出时运行某段代码。这里是一个确保一个打开的文件被关闭的例子:
```julia
f = open("file")
try
operate_on_file(f)
finally
close(f)
end
```
当控制流离开 `try` 代码块(例如,遇到 `return`,或者正常结束),`close(f)` 就会被执行。如果 `try` 代码块由于异常退出,这个异常会继续传递。`catch` 代码块可以和 `try` 还有 `finally` 配合使用。这时 `finally` 代码块会在 `catch` 处理错误之后才运行。
"""
kw"finally"
"""
break
立即跳出当前循环。
# 例子
```jldoctest
julia> i = 0
0
julia> while true
global i += 1
i > 5 && break
println(i)
end
1
2
3
4
5
```
"""
kw"break"
"""
continue
跳过当前循环迭代的剩余部分。
# 例子
```jldoctest
julia> for i = 1:6
iseven(i) && continue
println(i)
end
1
3
5
```
"""
kw"continue"
"""
do
创建一个匿名函数。例如:
```julia
map(1:10) do x
2x
end
```
等价于 `map(x->2x, 1:10)`。
像这样便可使用多个参数:
```julia
map(1:10, 11:20) do x, y
x + y
end
```
"""
kw"do"
"""
...
「splat」运算符 `...` 表示参数序列。`...` 可以在函数定义中用来表示该函数接受任意数量的参数。`...` 也可以用来将函数作用于参数序列。
# 例子
```jldoctest
julia> add(xs...) = reduce(+, xs)
add (generic function with 1 method)
julia> add(1, 2, 3, 4, 5)
15
julia> add([1, 2, 3]...)
6
julia> add(7, 1:100..., 1000:1100...)
111107
```
"""
kw"..."
"""
;
`;` 在 Julia 中具有与许多类 C 语言相似的作用,用于分隔前一个语句的结尾。`;` 在换行中不是必要的,但可以用于在单行中分隔语句或者将多个表达式连接为单个表达式。`;` 也用于抑制 REPL 和类似界面中的输出打印。
# 例子
```julia
julia> function foo()
x = "Hello, "; x *= "World!"
return x
end
foo (generic function with 1 method)
julia> bar() = (x = "Hello, Mars!"; return x)
bar (generic function with 1 method)
julia> foo();
julia> bar()
"Hello, Mars!"
```
"""
kw";"
"""
x && y
短路布尔 AND。
"""
kw"&&"
"""
x || y
短路布尔 OR。
"""
kw"||"
"""
ccall((function_name, library), returntype, (argtype1, ...), argvalue1, ...)
ccall(function_name, returntype, (argtype1, ...), argvalue1, ...)
ccall(function_pointer, returntype, (argtype1, ...), argvalue1, ...)
调用由 C 导出的共享库里的函数,该函数由元组 `(function_name, library)` 指定,其中的每个组件都是字符串或符号。若不指定库,还可以使用 `function_name` 的符号或字符串,它会在当前进程中解析。另外,`ccall` 也可用于调用函数指针 `function_pointer`,比如 `dlsym` 返回的函数指针。
请注意参数类型元组必须是字面上的元组,而不是元组类型的变量或表达式。
通过自动插入对 `unsafe_convert(argtype, cconvert(argtype, argvalue))` 的调用,每个传给 `ccall` 的 `argvalue` 将被类型转换为对应的 `argtype`。(有关的详细信息,请参阅 [`unsafe_convert`](@ref Base.unsafe_convert) 和 [`cconvert`](@ref Base.cconvert) 的文档。)在大多数情况下,这只会简单地调用 `convert(argtype, argvalue)`。
"""
kw"ccall"
"""
begin
`begin...end` 表示一个代码块。
```julia
begin
println("Hello, ")
println("World!")
end
```
通常,`begin` 不会是必需的,因为诸如 [`function`](@ref) and [`let`](@ref) 之类的关键字会隐式地开始代码块。另请参阅 [`;`](@ref)。
"""
kw"begin"
"""
struct
struct 是 Julia 中最常用的数据类型,由名称和一组字段指定。
```julia
struct Point
x
y
end
```
可对字段施加类型限制,该限制也可被参数化:
```julia
struct Point{X}
x::X
y::Float64
end
```
struct 可以通过 `<:` 语法声明一个抽象超类型:
A struct can also declare an abstract super type via `<:` syntax:
```julia
struct Point <: AbstractPoint
x
y
end
```
`struct` 默认是不可变的;这些类型的实例在构造后不能被修改。如需修改实例,请使用 [`mutable struct`](@ref) 来声明一个可以修改其实例的类型。
有关更多细节,比如怎么定义构造函数,请参阅手册的 [复合类型](@ref) 章节。
"""
kw"struct"
"""
mutable struct
`mutable struct` 类似于 [`struct`](@ref),但另外允许在构造后设置类型的字段。有关详细信息,请参阅 [复合类型](@ref)。
"""
kw"mutable struct"
"""
new
仅在内部构造函数中可用的特殊函数,用来创建该类型的对象。有关更多信息,请参阅手册的 [内部构造方法](@ref) 章节。
"""
kw"new"
"""
where
`where` 关键字创建一个类型,该类型是其他类型在一些变量上所有值的迭代并集。例如 `Vector{T} where T<:Real` 包含所有元素类型是某种 `Real` 的 [`Vector`](@ref)。
如果省略,变量上界默认为 `Any`:
```julia
Vector{T} where T # short for `where T<:Any`
```
变量也可以具有下界:
```julia
Vector{T} where T>:Int
Vector{T} where Int<:T<:Real
```
嵌套的 `where` 也有简洁的语法。例如,这行代码:
```julia
Pair{T, S} where S<:Array{T} where T<:Number
```
可以缩写为:
```julia
Pair{T, S} where {T<:Number, S<:Array{T}}
```
这种形式常见于方法签名:
请注意,在这种形式中,最外层变量列在最前面。这与使用语法 `T{p1, p2, ...}` 将类型「作用」于参数值时所替换变量的次序相匹配。
"""
kw"where"
"""
ans
一个引用最后一次计算结果的变量,在交互式提示符中会自动设置。
"""
kw"ans"
"""
Union{}
`Union{}`,即空的类型 [`Union`](@ref),是没有值的类型。也就是说,它具有决定性性质:对于任何 `x`,`isa(x, Union{}) == false`。`Base.Bottom` 被定义为其别名,`Union{}` 的类型是 `Core.TypeofBottom`。
# 例子
```jldoctest
julia> isa(nothing, Union{})
false
```
"""
kw"Union{}", Base.Bottom
"""
::
`::` 运算符用于类型声明,在程序中可被附加到表达式和变量后。详见手册的 [类型声明](@ref) 章节
在类型声明外,`::` 用于断言程序中的表达式和变量具有给定类型。
# 例子
```jldoctest
julia> (1+2)::AbstractFloat
ERROR: TypeError: typeassert: expected AbstractFloat, got Int64
julia> (1+2)::Int
3
```
"""
kw"::"
"""
Julia 的基础库。
"""
kw"Base"
| JuliaZH | https://github.com/JuliaCN/JuliaZH.jl.git |
|
[
"MIT"
] | 1.6.0 | a7dc7bfc2fc6638c18241bfcdb9d73c7dd256482 | code | 1186 | using REPL
using Base.Docs: catdoc, modules, DocStr, Binding, MultiDoc, isfield, namify, bindingexpr,
defined, resolve, getdoc, meta, aliasof, signature, isexpr
import Base.Docs: doc, formatdoc, parsedoc, apropos,
DocStr, lazy_iterpolate, quot, metadata, docexpr, keyworddoc
function REPL.lookup_doc(ex)
locale = get(ENV, "REPL_LOCALE", "en_US")
if locale == ""
locale == "en_US"
end
kw_dict = locale == "zh_CN" ? keywords : Docs.keywords
if haskey(kw_dict, ex)
Docs.parsedoc(kw_dict[ex])
elseif isa(ex, Union{Expr, Symbol})
binding = esc(bindingexpr(namify(ex)))
if isexpr(ex, :call) || isexpr(ex, :macrocall)
sig = esc(signature(ex))
:($(doc)($binding, $sig))
else
:($(doc)($binding))
end
else
:($(doc)($(typeof)($(esc(ex)))))
end
end
function Base.Docs.keyworddoc(__source__, __module__, str, def::Base.BaseDocs.Keyword)
@nospecialize str
docstr = esc(docexpr(__source__, __module__, lazy_iterpolate(str), metadata(__source__, __module__, def, false)))
return :($setindex!($(keywords), $docstr, $(esc(quot(def.name)))); nothing)
end
| JuliaZH | https://github.com/JuliaCN/JuliaZH.jl.git |
|
[
"MIT"
] | 1.6.0 | a7dc7bfc2fc6638c18241bfcdb9d73c7dd256482 | code | 271 | using Test
ENV["JULIA_PKG_SERVER"] = ""
using JuliaZH
@test !isempty(ENV["JULIA_PKG_SERVER"])
@testset "check ENV" begin
@test ENV["REPL_LOCALE"] == "zh_CN"
JuliaZH.set_mirror("BFSU")
@test ENV["JULIA_PKG_SERVER"] == "https://mirrors.bfsu.edu.cn/julia"
end
| JuliaZH | https://github.com/JuliaCN/JuliaZH.jl.git |
Subsets and Splits