text
stringlengths 4
5.48M
| meta
stringlengths 14
6.54k
|
---|---|
package org.elasticsearch.action.admin.indices.mapping.put;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import org.apache.logging.log4j.message.ParameterizedMessage;
import org.elasticsearch.action.ActionListener;
import org.elasticsearch.action.RequestValidators;
import org.elasticsearch.action.support.ActionFilters;
import org.elasticsearch.action.support.master.AcknowledgedResponse;
import org.elasticsearch.action.support.master.TransportMasterNodeAction;
import org.elasticsearch.cluster.ClusterState;
import org.elasticsearch.cluster.ack.ClusterStateUpdateResponse;
import org.elasticsearch.cluster.block.ClusterBlockException;
import org.elasticsearch.cluster.block.ClusterBlockLevel;
import org.elasticsearch.cluster.metadata.IndexNameExpressionResolver;
import org.elasticsearch.cluster.metadata.MetaDataMappingService;
import org.elasticsearch.cluster.service.ClusterService;
import org.elasticsearch.common.inject.Inject;
import org.elasticsearch.common.io.stream.StreamInput;
import org.elasticsearch.index.Index;
import org.elasticsearch.index.IndexNotFoundException;
import org.elasticsearch.tasks.Task;
import org.elasticsearch.threadpool.ThreadPool;
import org.elasticsearch.transport.TransportService;
import java.io.IOException;
import java.util.Objects;
import java.util.Optional;
/**
* Put mapping action.
*/
public class TransportPutMappingAction extends TransportMasterNodeAction<PutMappingRequest, AcknowledgedResponse> {
private static final Logger logger = LogManager.getLogger(TransportPutMappingAction.class);
private final MetaDataMappingService metaDataMappingService;
private final RequestValidators<PutMappingRequest> requestValidators;
@Inject
public TransportPutMappingAction(
final TransportService transportService,
final ClusterService clusterService,
final ThreadPool threadPool,
final MetaDataMappingService metaDataMappingService,
final ActionFilters actionFilters,
final IndexNameExpressionResolver indexNameExpressionResolver,
final RequestValidators<PutMappingRequest> requestValidators) {
super(PutMappingAction.NAME, transportService, clusterService, threadPool, actionFilters, PutMappingRequest::new,
indexNameExpressionResolver);
this.metaDataMappingService = metaDataMappingService;
this.requestValidators = Objects.requireNonNull(requestValidators);
}
@Override
protected String executor() {
// we go async right away
return ThreadPool.Names.SAME;
}
@Override
protected AcknowledgedResponse read(StreamInput in) throws IOException {
return new AcknowledgedResponse(in);
}
@Override
protected ClusterBlockException checkBlock(PutMappingRequest request, ClusterState state) {
String[] indices;
if (request.getConcreteIndex() == null) {
indices = indexNameExpressionResolver.concreteIndexNames(state, request);
} else {
indices = new String[] {request.getConcreteIndex().getName()};
}
return state.blocks().indicesBlockedException(ClusterBlockLevel.METADATA_WRITE, indices);
}
@Override
protected void masterOperation(Task task, final PutMappingRequest request, final ClusterState state,
final ActionListener<AcknowledgedResponse> listener) {
try {
final Index[] concreteIndices = request.getConcreteIndex() == null ?
indexNameExpressionResolver.concreteIndices(state, request)
: new Index[] {request.getConcreteIndex()};
final Optional<Exception> maybeValidationException = requestValidators.validateRequest(request, state, concreteIndices);
if (maybeValidationException.isPresent()) {
listener.onFailure(maybeValidationException.get());
return;
}
PutMappingClusterStateUpdateRequest updateRequest = new PutMappingClusterStateUpdateRequest()
.ackTimeout(request.timeout()).masterNodeTimeout(request.masterNodeTimeout())
.indices(concreteIndices).type(request.type())
.source(request.source());
metaDataMappingService.putMapping(updateRequest, new ActionListener<ClusterStateUpdateResponse>() {
@Override
public void onResponse(ClusterStateUpdateResponse response) {
listener.onResponse(new AcknowledgedResponse(response.isAcknowledged()));
}
@Override
public void onFailure(Exception t) {
logger.debug(() -> new ParameterizedMessage("failed to put mappings on indices [{}], type [{}]",
concreteIndices, request.type()), t);
listener.onFailure(t);
}
});
} catch (IndexNotFoundException ex) {
logger.debug(() -> new ParameterizedMessage("failed to put mappings on indices [{}], type [{}]",
request.indices(), request.type()), ex);
throw ex;
}
}
}
| {'content_hash': 'b3a3589723eb60f0b7d21b60a81ae707', 'timestamp': '', 'source': 'github', 'line_count': 117, 'max_line_length': 132, 'avg_line_length': 44.60683760683761, 'alnum_prop': 0.7116305805709906, 'repo_name': 'coding0011/elasticsearch', 'id': '4a9d833a29c547038943df45dafbfca668e2e809', 'size': '6007', 'binary': False, 'copies': '2', 'ref': 'refs/heads/master', 'path': 'server/src/main/java/org/elasticsearch/action/admin/indices/mapping/put/TransportPutMappingAction.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'ANTLR', 'bytes': '11081'}, {'name': 'Batchfile', 'bytes': '18064'}, {'name': 'Emacs Lisp', 'bytes': '3341'}, {'name': 'FreeMarker', 'bytes': '45'}, {'name': 'Groovy', 'bytes': '312193'}, {'name': 'HTML', 'bytes': '5519'}, {'name': 'Java', 'bytes': '41505710'}, {'name': 'Perl', 'bytes': '7271'}, {'name': 'Python', 'bytes': '55163'}, {'name': 'Shell', 'bytes': '119286'}]} |
package io.youi.upload
import java.io.File
case class UploadedFile(file: File, fileName: String) | {'content_hash': 'ac90536abbc95afe67de515e7a3f9c6d', 'timestamp': '', 'source': 'github', 'line_count': 5, 'max_line_length': 53, 'avg_line_length': 19.6, 'alnum_prop': 0.7959183673469388, 'repo_name': 'outr/youi', 'id': 'a2aff5b4a0c3649476b904330ff6c419d485d2b7', 'size': '98', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'app/jvm/src/main/scala/io/youi/upload/UploadedFile.scala', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '844'}, {'name': 'HTML', 'bytes': '6666'}, {'name': 'JavaScript', 'bytes': '106413'}, {'name': 'Scala', 'bytes': '3490560'}, {'name': 'Shell', 'bytes': '89'}]} |
package com.intel.analytics.bigdl.dllib.nn.tf
import com.intel.analytics.bigdl.dllib.tensor.Tensor
import com.intel.analytics.bigdl.dllib.utils.serializer.ModuleSerializationTest
import scala.util.Random
class NoOpSerialTest extends ModuleSerializationTest {
override def test(): Unit = {
val noOp = new com.intel.analytics.bigdl.dllib.nn.tf.NoOp[Float]().setName("noOp")
val input = Tensor[Float](5).apply1(_ => Random.nextFloat())
runSerializationTest(noOp, input)
}
}
| {'content_hash': '7148f7e3e1804024bb4cfec17c907d37', 'timestamp': '', 'source': 'github', 'line_count': 15, 'max_line_length': 86, 'avg_line_length': 32.733333333333334, 'alnum_prop': 0.7617107942973523, 'repo_name': 'intel-analytics/BigDL', 'id': '7205d95e929f5d17006d190502747f21447957e5', 'size': '1092', 'binary': False, 'copies': '2', 'ref': 'refs/heads/main', 'path': 'scala/dllib/src/test/scala/com/intel/analytics/bigdl/dllib/nn/tf/NoOpSpec.scala', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'C', 'bytes': '5342'}, {'name': 'Dockerfile', 'bytes': '139304'}, {'name': 'Java', 'bytes': '1321348'}, {'name': 'Jupyter Notebook', 'bytes': '54112822'}, {'name': 'Lua', 'bytes': '1904'}, {'name': 'Makefile', 'bytes': '19253'}, {'name': 'PowerShell', 'bytes': '1137'}, {'name': 'PureBasic', 'bytes': '593'}, {'name': 'Python', 'bytes': '8825782'}, {'name': 'RobotFramework', 'bytes': '16117'}, {'name': 'Scala', 'bytes': '13216148'}, {'name': 'Shell', 'bytes': '848241'}]} |
package org.apache.ambari.eventdb.db;
import java.io.IOException;
import java.util.List;
import org.apache.ambari.eventdb.model.DataTable;
import org.apache.ambari.eventdb.model.Jobs.JobDBEntry;
import org.apache.ambari.eventdb.model.TaskAttempt;
import org.apache.ambari.eventdb.model.WorkflowContext;
import org.apache.ambari.eventdb.model.Workflows;
import org.apache.ambari.eventdb.model.Workflows.WorkflowDBEntry.WorkflowFields;
public interface DBConnector {
public void submitJob(JobDBEntry j, WorkflowContext context) throws IOException;
public void updateJob(JobDBEntry j) throws IOException;
public Workflows fetchWorkflows() throws IOException;
public Workflows fetchWorkflows(WorkflowFields field, boolean sortAscending, int offset, int limit) throws IOException;
public DataTable fetchWorkflows(int offset, int limit, String searchTerm, int echo, WorkflowFields field, boolean sortAscending, String searchWorkflowId,
String searchWorkflowName, String searchWorkflowType, String searchUserName, int minJobs, int maxJobs, long minInputBytes, long maxInputBytes,
long minOutputBytes, long maxOutputBytes, long minDuration, long maxDuration, long minStartTime, long maxStartTime, long minFinishTime, long maxFinishTime)
throws IOException;
public List<JobDBEntry> fetchJobDetails(String workflowID) throws IOException;
public List<JobDBEntry> fetchJobDetails(long minFinishTime, long maxStartTime) throws IOException;
public long[] fetchJobStartStopTimes(String jobID) throws IOException;
public List<TaskAttempt> fetchJobTaskAttempts(String jobID) throws IOException;
public List<TaskAttempt> fetchWorkflowTaskAttempts(String workflowID) throws IOException;
public List<TaskAttempt> fetchTaskAttempts(long minFinishTime, long maxStartTime) throws IOException;
public void close();
}
| {'content_hash': '5cf50d7d81438c8d9bce7303e7cc5673', 'timestamp': '', 'source': 'github', 'line_count': 41, 'max_line_length': 161, 'avg_line_length': 45.65853658536585, 'alnum_prop': 0.8125, 'repo_name': 'zouzhberk/ambaridemo', 'id': 'b859114950c94d18402d253bed1ab486db79c0e1', 'size': '2674', 'binary': False, 'copies': '3', 'ref': 'refs/heads/master', 'path': 'demo-server/src/main/java/org/apache/ambari/eventdb/db/DBConnector.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Batchfile', 'bytes': '5982'}, {'name': 'Groff', 'bytes': '13935'}, {'name': 'HTML', 'bytes': '52'}, {'name': 'Java', 'bytes': '8681846'}, {'name': 'PLSQL', 'bytes': '2160'}, {'name': 'PLpgSQL', 'bytes': '105599'}, {'name': 'PowerShell', 'bytes': '43170'}, {'name': 'Python', 'bytes': '2751909'}, {'name': 'Ruby', 'bytes': '9652'}, {'name': 'SQLPL', 'bytes': '2117'}, {'name': 'Shell', 'bytes': '247846'}]} |
import React, { Component } from 'react'
import globalConfig from '../../config'
import icons from '../../icons/css/icons.css'
import { fetchTaxonomies } from '../../core/firebaseRestAPI'
import { taxonomiesWithIcons } from '../../lib/taxonomies'
import Home from '../../components/Home'
import Layout from '../../components/Layout'
import Loading from '../../components/Loading'
class HomePage extends Component {
constructor(props) {
super(props)
this.state = {
taxonomies: [],
}
}
componentDidMount() {
fetchTaxonomies()
.then(taxonomies => {
this.setState({
taxonomies: taxonomiesWithIcons(taxonomies)
})
})
document.title = globalConfig.title
}
render() {
const { taxonomies } = this.state
return (
<Layout>
{ taxonomies.length ? <Home categories={taxonomies} /> : <Loading /> }
</Layout>
)
}
}
export default HomePage
| {'content_hash': '9cba32a1642589faf64535589ac7fe60', 'timestamp': '', 'source': 'github', 'line_count': 43, 'max_line_length': 78, 'avg_line_length': 21.88372093023256, 'alnum_prop': 0.6216790648246546, 'repo_name': 'zendesk/copenhelp', 'id': 'c6a54fb36d926f56ac818ade7fefa8391d257639', 'size': '941', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'pages/home/index.js', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'CSS', 'bytes': '26174'}, {'name': 'HTML', 'bytes': '1189'}, {'name': 'JavaScript', 'bytes': '159181'}, {'name': 'Shell', 'bytes': '1308'}]} |
require_relative 'configuration'
require 'active_support/core_ext/class/attribute'
require 'hashie'
module Wprapper
class Base < Hashie::Dash
class_attribute :configuration
self.configuration = Configuration.current
class << self
def wordpress
Wordpress.new(configuration)
end
def wordpress_json_api
WordpressJsonApi.new(configuration)
end
end
end
end
| {'content_hash': 'eb47d744aecd4bcf3f003fe16b466842', 'timestamp': '', 'source': 'github', 'line_count': 21, 'max_line_length': 49, 'avg_line_length': 19.80952380952381, 'alnum_prop': 0.6995192307692307, 'repo_name': 'doublewide/wprapper', 'id': '4604ca347fc5d23e38dbfd8ffaf0ae1768fa323f', 'size': '416', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'lib/wprapper/base.rb', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Ruby', 'bytes': '23048'}]} |
<?xml version="1.0" encoding="utf-8"?>
<inset android:insetLeft="3.0dip" android:insetRight="3.0dip" android:insetTop="10.0dip" android:insetBottom="10.0dip"
xmlns:android="http://schemas.android.com/apk/res/android">
<shape android:shape="rectangle">
<corners android:radius="1.0dip" />
<solid android:color="#1a000000" />
<stroke android:width="1.0px" android:color="#66fafafa" />
</shape>
</inset> | {'content_hash': 'fb8781c7985d10066a5aea5a47392c04', 'timestamp': '', 'source': 'github', 'line_count': 9, 'max_line_length': 118, 'avg_line_length': 48.22222222222222, 'alnum_prop': 0.6705069124423964, 'repo_name': 'BatMan-Rom/ModdedFiles', 'id': '8bedd70c6cefb85fba7532a7f3b9cd537f388ba8', 'size': '434', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'SecSettings/res/drawable/tw_btn_background_enabled.xml', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'GLSL', 'bytes': '15069'}, {'name': 'HTML', 'bytes': '139176'}, {'name': 'Smali', 'bytes': '541934400'}]} |
$(document).ready(function() {
// Load modal typeahead when button pushed to add Conference
$('body').on('click', '#add-new-conference', function(){
startTypeAhead();
})
// Activate datepicker widget
$('#id_date_begins').datepicker({
dateFormat: 'yy-mm-dd'
});
// Show modal on load if form errors
var newConferenceFormHasErrors = $('#new-conference-form-has-errors').val();
if (newConferenceFormHasErrors == 'true'){
$('#modal-quick-add-event').modal('show');
};
// Update assignments after drag/drop of users
function updateAssignments(userId, receiverId){
var confId = $('#id_event').val();
$.ajax({
url: '/crm/update_user_assignments/',
type: 'POST',
data: {
'conf_id': confId,
'user_id': userId,
'role': receiverId,
}
});
};
// Activate Drag Drop Sortation (called after AJAX load)
function startDragDrop() {
$('.connectedSortable').sortable({
connectWith: '.connectedSortable',
receive: function(event, ui){
var movedId = ui.item.attr('user-id');
var movedItem = ui.item;
var receiverId = $(this).attr('id');
var sender = ui.sender;
var receiver = $(this);
updateAssignments(movedId, receiverId);
}
}).disableSelection();
};
// Select conference and load selection widget
$('body').on('click', '#select-conference', function(){
var confId = $('#id_event').val();
$.ajax({
url: '/crm/create_selection_widget/' + confId + '/',
type: 'GET',
// data: {
// 'conf_id': confId,
// },
success: function(data){
$('#selection-widget').html(data);
startDragDrop();
startTypeAhead();
}
});
});
// Change staff member's method of list generation (filter vs new)
$('body').on('click', '#btn-change-filter', function(){
var filterMaster = $('input[name=filter_master_selects]:checked').val();
var staffId = $('#active-staff-id').val();
callStaffMemberTerritoryDetails(staffId, filterMaster);
});
// Add general list selection and update relevant parts of page
$('body').on('click', '#master #btn-add-new-select', function(){
var confId = $('#id_event').val();
var includeExclude = $('#master #id_include_exclude').val();
var mainCategory = $('#master #id_main_category').val();
var mainCategory2 = $('#master #id_main_category2').val();
var geo = $('#master #id_geo').val();
var industry = $('#master #id_industry').val();
var company = $('#master #id_company').val();
var dept = $('#master #id_dept').val();
var title = $('#master #id_title').val();
if (mainCategory || geo || industry || company || dept || title) {
$.ajax({
url: '/crm/add_master_list_select/',
type: 'POST',
data: {
'conf_id': confId,
'include_exclude': includeExclude,
'main_category': mainCategory,
'main_category2': mainCategory2,
'geo': geo,
'industry': industry,
'company': company,
'dept': dept,
'title': title,
},
success: function(data){
$('#master').html(data);
startTypeAhead();
}
});
};
});
// Add personal list selection and update relevant parts of page
$('body').on('click', '#btn-add-new-personal-select', function(){
var confId = $('#id_event').val();
var staffId = $('#active-staff-id').val();
var includeExclude = $('#personal-select-details #id_include_exclude').val();
var mainCategory = $('#personal-select-details #id_main_category').val();
var mainCategory2 = $('#personal-select-details #id_main_category2').val();
var geo = $('#personal-select-details #id_geo').val();
var industry = $('#personal-select-details #id_industry').val();
var company = $('#personal-select-details #id_company').val();
var dept = $('#personal-select-details #id_dept').val();
var division1 = $('#personal-select-details #id_division1').val();
var division2 = $('#personal-select-details #id_division2').val();
var title = $('#personal-select-details #id_title').val();
if (mainCategory || geo || industry || company || dept || division1 || division2 || title || mainCategory2) {
$.ajax({
url: '/crm/add_personal_list_select/',
type: 'POST',
data: {
'conf_id': confId,
'staff_id': staffId,
'include_exclude': includeExclude,
'main_category': mainCategory,
'main_category2': mainCategory2,
'geo': geo,
'industry': industry,
'company': company,
'dept': dept,
'division1': division1,
'division2': division2,
'title': title,
},
success: function(data){
$('#person-select-options').html(data);
startTypeAhead();
}
});
};
});
// Delete general list selection and update relevant parts of page
$('body').on('click', '.btn-delete-master-select', function(){
var confId = $('#id_event').val();
var staffId = $('#active-staff-id').val();
var selectId = $(this).attr('select-value');
$.ajax({
url: '/crm/delete_master_list_select/',
type: 'POST',
data: {
'conf_id': confId,
'select_id': selectId,
'staff_id': staffId,
},
success: function(data){
$('#master').html(data);
startTypeAhead();
}
});
});
// Delete personal list selection and update relevant parts of page
$('body').on('click', '.btn-delete-personal-select', function(){
var confId = $('#id_event').val();
var selectId = $(this).attr('select-value');
var staffId = $('#active-staff-id').val();
$.ajax({
url: '/crm/delete_personal_list_select/',
type: 'POST',
data: {
'conf_id': confId,
'select_id': selectId,
'staff_id': staffId,
},
success: function(data){
$('#person-select-options').html(data);
startTypeAhead();
}
});
});
// typeahead autocomplete for dept, industry & company fields
var deptSuggestionClass = new Bloodhound({
limit: 20,
datumTokenizer: Bloodhound.tokenizers.obj.whitespace('value'),
queryTokenizer: Bloodhound.tokenizers.whitespace,
remote: {
url: '/crm/suggest_dept/',
replace: function(url, query){
return url + "?q=" + query;
},
filter: function(my_Suggestion_class) {
return $.map(my_Suggestion_class, function(data){
return {value: data.identifier};
});
}
}
});
deptSuggestionClass.initialize();
var companySuggestionClass = new Bloodhound({
limit: 20,
datumTokenizer: Bloodhound.tokenizers.obj.whitespace('value'),
queryTokenizer: Bloodhound.tokenizers.whitespace,
remote: {
url: '/crm/suggest_company/',
replace: function(url, query){
return url + "?q=" + query;
},
filter: function(my_Suggestion_class) {
return $.map(my_Suggestion_class, function(data){
return {value: data.identifier};
});
}
}
});
companySuggestionClass.initialize();
var industrySuggestionClass = new Bloodhound({
limit: 20,
datumTokenizer: Bloodhound.tokenizers.obj.whitespace('value'),
queryTokenizer: Bloodhound.tokenizers.whitespace,
remote: {
url: '/crm/suggest_industry/',
replace: function(url, query){
return url + "?q=" + query;
},
filter: function(my_Suggestion_class) {
return $.map(my_Suggestion_class, function(data){
return {value: data.identifier};
});
}
}
});
industrySuggestionClass.initialize();
// function that will start the typeahead (needs to be called after each ajax)
function startTypeAhead(){
$('#master #id_dept, #person-select-options #id_dept, #id_default_dept').typeahead({
hint: true,
highlight: true,
minLength: 1
},
{
name: 'value',
displayKey: 'value',
source: deptSuggestionClass.ttAdapter(),
templates: {
empty: [
'<div class="tt-suggestion">',
'No Items Found',
'</div>'
].join('\n')
}
});
$('#master #id_company, #person-select-options #id_company').typeahead({
hint: true,
highlight: true,
minLength: 2
},
{
name: 'value',
displayKey: 'value',
source: companySuggestionClass.ttAdapter(),
templates: {
empty: [
'<div class="tt-suggestion">',
'No Items Found',
'</div>'
].join('\n')
}
});
$('#master #id_industry, #person-select-options #id_industry').typeahead({
hint: true,
highlight: true,
minLength: 2
},
{
name: 'value',
displayKey: 'value',
source: industrySuggestionClass.ttAdapter(),
templates: {
empty: [
'<div class="tt-suggestion">',
'No Items Found',
'</div>'
].join('\n')
}
});
};
// load appropriate staff GROUP content into personal select panel
$('body').on('click', '.staff-select', function(){
var sectionChosen = $(this).attr('select-key');
var confId = $('#id_event').val();
$.ajax({
url: '/crm/load_staff_category_selects/',
type: 'GET',
data: {
'conf_id': confId,
'section_chosen': sectionChosen,
},
success: function(data){
$('#personal-selects').html(data);
var numStaff = $('.btn-staff-select', data).length;
if (numStaff == 1){
var staffId = $('.btn-staff-select', data).attr('staff-id');
callStaffMemberTerritoryDetails(staffId);
}
startTypeAhead();
}
})
});
// function to do an ajax load of individual staff member select page
function callStaffMemberTerritoryDetails(staffId, filterSwitch){
var confId = $('#id_event').val();
var ajaxData = {
'event_id': confId,
'user_id': staffId,
};
if (filterSwitch !== undefined) {
ajaxData['filter_switch'] = filterSwitch;
};
$.ajax({
url: '/crm/load_staff_member_selects/',
type: 'GET',
data: ajaxData,
success: function(data){
$('#personal-select-details').html(data);
startTypeAhead();
}
});
};
// load appropriate staff MEMBER content into personal select panel
$('body').on('click', '.btn-staff-select', function(){
$('.btn-staff-select').not(this).each(function(){
$(this).removeClass('btn-primary');
$(this).addClass('btn-default');
});
$(this).removeClass('btn-default');
$(this).addClass('btn-primary');
var staffId = $(this).attr('staff-id');
callStaffMemberTerritoryDetails(staffId);
})
});
| {'content_hash': '9a2dc54af2e7a97f1bce350b03fb58fa', 'timestamp': '', 'source': 'github', 'line_count': 367, 'max_line_length': 113, 'avg_line_length': 30.28882833787466, 'alnum_prop': 0.5556854983807125, 'repo_name': 'asterix135/infonex_crm', 'id': '2bdf5e31e5bf655559b7ea30b4a15686e2e1425d', 'size': '11161', 'binary': False, 'copies': '2', 'ref': 'refs/heads/master', 'path': 'crm/static/crm/javascript/manage_territory.js', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '112273'}, {'name': 'HTML', 'bytes': '272534'}, {'name': 'JavaScript', 'bytes': '415610'}, {'name': 'Python', 'bytes': '674129'}, {'name': 'Shell', 'bytes': '1837'}]} |
#pragma hdrstop
#endif
#include "wx/region.h"
#ifndef WX_PRECOMP
#include "wx/gdicmn.h"
#endif
#include "wx/msw/private.h"
wxIMPLEMENT_DYNAMIC_CLASS(wxRegion, wxGDIObject);
wxIMPLEMENT_DYNAMIC_CLASS(wxRegionIterator, wxObject);
// ----------------------------------------------------------------------------
// wxRegionRefData implementation
// ----------------------------------------------------------------------------
class WXDLLEXPORT wxRegionRefData : public wxGDIRefData
{
public:
wxRegionRefData()
{
m_region = 0;
}
wxRegionRefData(const wxRegionRefData& data) : wxGDIRefData()
{
#if defined(__WIN32__) && !defined(__WXMICROWIN__) && !defined(__WXWINCE__)
DWORD noBytes = ::GetRegionData(data.m_region, 0, NULL);
RGNDATA *rgnData = (RGNDATA*) new char[noBytes];
::GetRegionData(data.m_region, noBytes, rgnData);
m_region = ::ExtCreateRegion(NULL, noBytes, rgnData);
delete[] (char*) rgnData;
#else
RECT rect;
::GetRgnBox(data.m_region, &rect);
m_region = ::CreateRectRgnIndirect(&rect);
#endif
}
virtual ~wxRegionRefData()
{
::DeleteObject(m_region);
m_region = 0;
}
HRGN m_region;
private:
// Cannot use
// wxDECLARE_NO_COPY_CLASS(wxRegionRefData);
// because copy constructor is explicitly declared above;
// but no copy assignment operator is defined, so declare
// it private to prevent the compiler from defining it:
wxRegionRefData& operator=(const wxRegionRefData&);
};
#define M_REGION (((wxRegionRefData*)m_refData)->m_region)
#define M_REGION_OF(rgn) (((wxRegionRefData*)(rgn.m_refData))->m_region)
// ============================================================================
// wxRegion implementation
// ============================================================================
// ----------------------------------------------------------------------------
// ctors and dtor
// ----------------------------------------------------------------------------
wxRegion::wxRegion()
{
m_refData = NULL;
}
wxRegion::wxRegion(WXHRGN hRegion)
{
m_refData = new wxRegionRefData;
M_REGION = (HRGN) hRegion;
}
wxRegion::wxRegion(wxCoord x, wxCoord y, wxCoord w, wxCoord h)
{
m_refData = new wxRegionRefData;
M_REGION = ::CreateRectRgn(x, y, x + w, y + h);
}
wxRegion::wxRegion(const wxPoint& topLeft, const wxPoint& bottomRight)
{
m_refData = new wxRegionRefData;
M_REGION = ::CreateRectRgn(topLeft.x, topLeft.y, bottomRight.x, bottomRight.y);
}
wxRegion::wxRegion(const wxRect& rect)
{
m_refData = new wxRegionRefData;
M_REGION = ::CreateRectRgn(rect.x, rect.y, rect.x + rect.width, rect.y + rect.height);
}
wxRegion::wxRegion(size_t n, const wxPoint *points, wxPolygonFillMode fillStyle)
{
#if defined(__WXMICROWIN__) || defined(__WXWINCE__)
wxUnusedVar(n);
wxUnusedVar(points);
wxUnusedVar(fillStyle);
m_refData = NULL;
M_REGION = NULL;
#else
m_refData = new wxRegionRefData;
M_REGION = ::CreatePolygonRgn
(
(POINT*)points,
n,
fillStyle == wxODDEVEN_RULE ? ALTERNATE : WINDING
);
#endif
}
wxRegion::~wxRegion()
{
// m_refData unrefed in ~wxObject
}
wxGDIRefData *wxRegion::CreateGDIRefData() const
{
return new wxRegionRefData;
}
wxGDIRefData *wxRegion::CloneGDIRefData(const wxGDIRefData *data) const
{
return new wxRegionRefData(*(wxRegionRefData *)data);
}
// ----------------------------------------------------------------------------
// wxRegion operations
// ----------------------------------------------------------------------------
// Clear current region
void wxRegion::Clear()
{
UnRef();
}
bool wxRegion::DoOffset(wxCoord x, wxCoord y)
{
wxCHECK_MSG( GetHrgn(), false, wxT("invalid wxRegion") );
if ( !x && !y )
{
// nothing to do
return true;
}
AllocExclusive();
if ( ::OffsetRgn(GetHrgn(), x, y) == ERROR )
{
wxLogLastError(wxT("OffsetRgn"));
return false;
}
return true;
}
// combine another region with this one
bool wxRegion::DoCombine(const wxRegion& rgn, wxRegionOp op)
{
// we can't use the API functions if we don't have a valid region handle
if ( !m_refData )
{
// combining with an empty/invalid region works differently depending
// on the operation
switch ( op )
{
case wxRGN_COPY:
case wxRGN_OR:
case wxRGN_XOR:
*this = rgn;
break;
default:
wxFAIL_MSG( wxT("unknown region operation") );
// fall through
case wxRGN_AND:
case wxRGN_DIFF:
// leave empty/invalid
return false;
}
}
else // we have a valid region
{
AllocExclusive();
int mode;
switch ( op )
{
case wxRGN_AND:
mode = RGN_AND;
break;
case wxRGN_OR:
mode = RGN_OR;
break;
case wxRGN_XOR:
mode = RGN_XOR;
break;
case wxRGN_DIFF:
mode = RGN_DIFF;
break;
default:
wxFAIL_MSG( wxT("unknown region operation") );
// fall through
case wxRGN_COPY:
mode = RGN_COPY;
break;
}
if ( ::CombineRgn(M_REGION, M_REGION, M_REGION_OF(rgn), mode) == ERROR )
{
wxLogLastError(wxT("CombineRgn"));
return false;
}
}
return true;
}
// ----------------------------------------------------------------------------
// wxRegion bounding box
// ----------------------------------------------------------------------------
// Outer bounds of region
bool wxRegion::DoGetBox(wxCoord& x, wxCoord& y, wxCoord&w, wxCoord &h) const
{
if (m_refData)
{
RECT rect;
::GetRgnBox(M_REGION, & rect);
x = rect.left;
y = rect.top;
w = rect.right - rect.left;
h = rect.bottom - rect.top;
return true;
}
else
{
x = y = w = h = 0;
return false;
}
}
// Is region empty?
bool wxRegion::IsEmpty() const
{
wxCoord x, y, w, h;
GetBox(x, y, w, h);
return (w == 0) && (h == 0);
}
bool wxRegion::DoIsEqual(const wxRegion& region) const
{
return ::EqualRgn(M_REGION, M_REGION_OF(region)) != 0;
}
// ----------------------------------------------------------------------------
// wxRegion hit testing
// ----------------------------------------------------------------------------
// Does the region contain the point (x,y)?
wxRegionContain wxRegion::DoContainsPoint(wxCoord x, wxCoord y) const
{
if (!m_refData)
return wxOutRegion;
return ::PtInRegion(M_REGION, (int) x, (int) y) ? wxInRegion : wxOutRegion;
}
// Does the region contain the rectangle (x, y, w, h)?
wxRegionContain wxRegion::DoContainsRect(const wxRect& rect) const
{
if (!m_refData)
return wxOutRegion;
RECT rc;
wxCopyRectToRECT(rect, rc);
return ::RectInRegion(M_REGION, &rc) ? wxInRegion : wxOutRegion;
}
// Get internal region handle
WXHRGN wxRegion::GetHRGN() const
{
return (WXHRGN)(m_refData ? M_REGION : 0);
}
// ============================================================================
// wxRegionIterator implementation
// ============================================================================
// ----------------------------------------------------------------------------
// wxRegionIterator ctors/dtor
// ----------------------------------------------------------------------------
void wxRegionIterator::Init()
{
m_current =
m_numRects = 0;
m_rects = NULL;
}
wxRegionIterator::~wxRegionIterator()
{
delete [] m_rects;
}
// Initialize iterator for region
wxRegionIterator::wxRegionIterator(const wxRegion& region)
{
m_rects = NULL;
Reset(region);
}
wxRegionIterator& wxRegionIterator::operator=(const wxRegionIterator& ri)
{
delete [] m_rects;
m_current = ri.m_current;
m_numRects = ri.m_numRects;
if ( m_numRects )
{
m_rects = new wxRect[m_numRects];
for ( long n = 0; n < m_numRects; n++ )
m_rects[n] = ri.m_rects[n];
}
else
{
m_rects = NULL;
}
return *this;
}
// ----------------------------------------------------------------------------
// wxRegionIterator operations
// ----------------------------------------------------------------------------
// Reset iterator for a new region.
void wxRegionIterator::Reset(const wxRegion& region)
{
m_current = 0;
m_region = region;
wxDELETEA(m_rects);
if (m_region.Empty())
m_numRects = 0;
else
{
DWORD noBytes = ::GetRegionData(((wxRegionRefData*)region.m_refData)->m_region, 0, NULL);
RGNDATA *rgnData = (RGNDATA*) new char[noBytes];
::GetRegionData(((wxRegionRefData*)region.m_refData)->m_region, noBytes, rgnData);
RGNDATAHEADER* header = (RGNDATAHEADER*) rgnData;
m_rects = new wxRect[header->nCount];
RECT* rect = (RECT*) ((char*)rgnData + sizeof(RGNDATAHEADER));
size_t i;
for (i = 0; i < header->nCount; i++)
{
m_rects[i] = wxRect(rect->left, rect->top,
rect->right - rect->left, rect->bottom - rect->top);
rect ++; // Advances pointer by sizeof(RECT)
}
m_numRects = header->nCount;
delete[] (char*) rgnData;
}
}
wxRegionIterator& wxRegionIterator::operator++()
{
if (m_current < m_numRects)
++m_current;
return *this;
}
wxRegionIterator wxRegionIterator::operator ++ (int)
{
wxRegionIterator tmp = *this;
if (m_current < m_numRects)
++m_current;
return tmp;
}
// ----------------------------------------------------------------------------
// wxRegionIterator accessors
// ----------------------------------------------------------------------------
wxCoord wxRegionIterator::GetX() const
{
wxCHECK_MSG( m_current < m_numRects, 0, wxT("invalid wxRegionIterator") );
return m_rects[m_current].x;
}
wxCoord wxRegionIterator::GetY() const
{
wxCHECK_MSG( m_current < m_numRects, 0, wxT("invalid wxRegionIterator") );
return m_rects[m_current].y;
}
wxCoord wxRegionIterator::GetW() const
{
wxCHECK_MSG( m_current < m_numRects, 0, wxT("invalid wxRegionIterator") );
return m_rects[m_current].width;
}
wxCoord wxRegionIterator::GetH() const
{
wxCHECK_MSG( m_current < m_numRects, 0, wxT("invalid wxRegionIterator") );
return m_rects[m_current].height;
}
| {'content_hash': 'd2dec78a4a91f715ef3a82e6faebe0be', 'timestamp': '', 'source': 'github', 'line_count': 440, 'max_line_length': 97, 'avg_line_length': 24.570454545454545, 'alnum_prop': 0.5053186569235039, 'repo_name': 'emoon/prodbg-third-party', 'id': 'b1dff4e9466df4efae900584f4c2e9d0bd11d028', 'size': '11673', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/wxWidgets/src/msw/region.cpp', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'AppleScript', 'bytes': '10241'}, {'name': 'Assembly', 'bytes': '16038'}, {'name': 'Awk', 'bytes': '42674'}, {'name': 'Batchfile', 'bytes': '32509'}, {'name': 'C', 'bytes': '7545991'}, {'name': 'C++', 'bytes': '39378425'}, {'name': 'CMake', 'bytes': '20962'}, {'name': 'CSS', 'bytes': '4659'}, {'name': 'DIGITAL Command Language', 'bytes': '41524'}, {'name': 'Groff', 'bytes': '479741'}, {'name': 'HTML', 'bytes': '1110831'}, {'name': 'Inno Setup', 'bytes': '6562'}, {'name': 'JavaScript', 'bytes': '1375'}, {'name': 'Lua', 'bytes': '7205'}, {'name': 'Makefile', 'bytes': '100395'}, {'name': 'Module Management System', 'bytes': '226048'}, {'name': 'Nemerle', 'bytes': '28217'}, {'name': 'Objective-C', 'bytes': '5572961'}, {'name': 'Objective-C++', 'bytes': '818606'}, {'name': 'Perl', 'bytes': '33572'}, {'name': 'Python', 'bytes': '108744'}, {'name': 'R', 'bytes': '672260'}, {'name': 'Rebol', 'bytes': '1286'}, {'name': 'Shell', 'bytes': '1074413'}, {'name': 'TeX', 'bytes': '5028'}, {'name': 'XSLT', 'bytes': '44320'}]} |
module.exports = function(sql){
//model
this.ideaModel = require('../model/ideaModel');
/**
* @method selectIdeasBellow
* @param user_id
* @param n Number of ideas to be selected
* @param before_id If null, takes the last ideas
* @param callback(ideaModel[])
**/
this.selectIdeasBellow = function(user_id,n,bellow_of_id,callback)
{
sql.query('CALL mindsurf.SELECT_IDEAS_BELLOW('+user_id+','+n+','+bellow_of_id+')', function(err, rows, fields)
{
if (err) throw err;
else callback(rows[0]);
});
}
/**
* @method selectIdeasOntop
* @param user_id
* @param ontop_of_id
* @param callback(ideaModel[])
**/
this.selectIdeasOntop = function(user_id,ontop_of_id,callback)
{
sql.query('CALL mindsurf.SELECT_IDEAS_ONTOP('+user_id+','+ontop_of_id+')', function(err, rows, fields)
{
if (err) throw err;
else callback(rows[0]);
});
}
/**
* @method insertIdea
* @param user_id
* @param idea
* @param reference
* @param tag_id
* @param callback()
**/
this.insertIdea = function(user_id,idea,reference,tag_id,callback)
{
sql.query('CALL mindsurf.INSERT_IDEA('+user_id+',"'+idea+'","'+reference+'",'+tag_id+')', function(err, rows, fields)
{
if (err) throw err;
else callback();
});
}
return this;
}
| {'content_hash': '90b2a223eafc6befa1b8eeda6f60ca19', 'timestamp': '', 'source': 'github', 'line_count': 54, 'max_line_length': 125, 'avg_line_length': 27.685185185185187, 'alnum_prop': 0.5377926421404682, 'repo_name': 'mindsurf/services', 'id': '76505c54a9196aa55b05f5de623eccbe6dc07e54', 'size': '1495', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'data/dao/ideaDao.js', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'JavaScript', 'bytes': '19749'}]} |
#ifndef __VCGLIB_IMPORTERDAE
#define __VCGLIB_IMPORTERDAE
//importer for collada's files
#include <wrap/dae/util_dae.h>
#include <wrap/dae/poly_triangulator.h>
// uncomment one of the following line to enable the Verbose debugging for the parsing
//#define QDEBUG if(1) ; else {assert(0);}
#define QDEBUG qDebug
namespace vcg {
namespace tri {
namespace io {
template<typename OpenMeshType>
class ImporterDAE : public UtilDAE
{
public:
class ColladaFace;
class ColladaVertex;
class ColladaTypes: public vcg::UsedTypes < vcg::Use<ColladaVertex>::template AsVertexType,
vcg::Use<ColladaFace >::template AsFaceType >{};
class ColladaVertex : public vcg::Vertex< ColladaTypes,
vcg::vertex::Coord3f, /* 12b */
vcg::vertex::BitFlags, /* 4b */
vcg::vertex::Normal3f, /* 12b */
vcg::vertex::Color4b /* 4b */
> {};
class ColladaFace : public vcg::Face< ColladaTypes,
vcg::face::VertexRef, /*12b */
vcg::face::BitFlags, /* 4b */
vcg::face::Normal3f, /*12b */
vcg::face::Color4b, /* 0b */
vcg::face::WedgeTexCoord2f /* 0b */
> {};
class ColladaMesh : public vcg::tri::TriMesh< std::vector<ColladaVertex>, std::vector<ColladaFace> > {};
private:
static int WedgeNormalAttribute(ColladaMesh& m,const QStringList face,const QStringList wn,const QDomNode wnsrc,const int meshfaceind,const int faceind,const int component)
{
int indnm = -1;
if (!wnsrc.isNull())
{
indnm = face.at(faceind).toInt();
assert(indnm * 3 < wn.size());
m.face[meshfaceind].WN(component) = vcg::Point3f(wn.at(indnm * 3).toFloat(),wn.at(indnm * 3 + 1).toFloat(),wn.at(indnm * 3 + 2).toFloat());
}
return indnm;
}
static int WedgeTextureAttribute(ColladaMesh& m,const QStringList face,int ind_txt,const QStringList wt,const QDomNode wtsrc,const int meshfaceind,const int faceind,const int component,const int stride = 2)
{
int indtx = -1;
if (!wtsrc.isNull())
{
indtx = face.at(faceind).toInt();
//int num = wt.size();
assert(indtx * stride < wt.size());
m.face[meshfaceind].WT(component) = vcg::TexCoord2<float>();
m.face[meshfaceind].WT(component).U() = wt.at(indtx * stride).toFloat();
m.face[meshfaceind].WT(component).V() = wt.at(indtx * stride + 1).toFloat();
m.face[meshfaceind].WT(component).N() = ind_txt;
}
return indtx;
}
// this one is used for the polylist nodes
static int WedgeTextureAttribute(typename ColladaMesh::FaceType::TexCoordType & WT, const QStringList faceIndexList, int ind_txt, const QStringList wt, const QDomNode wtsrc,const int faceind,const int stride = 2)
{
int indtx = -1;
if (!wtsrc.isNull())
{
indtx = faceIndexList.at(faceind).toInt();
//int num = wt.size();
assert(indtx * stride < wt.size());
WT = vcg::TexCoord2<float>();
WT.U() = wt.at(indtx * stride).toFloat();
WT.V() = wt.at(indtx * stride + 1).toFloat();
WT.N() = ind_txt;
}
return indtx;
}
static int VertexColorAttribute(ColladaMesh& m,const QStringList face,const QStringList wc,const QDomNode wcsrc,const int faceind, const int vertind,const int colorcomponent)
{
int indcl = -1;
if (!wcsrc.isNull())
{
indcl = face.at(faceind).toInt();
assert((colorcomponent == 4) || (colorcomponent == 3));
assert(indcl * colorcomponent < wc.size());
vcg::Color4b c;
if (colorcomponent == 3)
c[3] = 255;
for(unsigned int ii = 0;ii < colorcomponent;++ii)
c[ii] = (unsigned char)(wc.at(indcl * colorcomponent + ii).toFloat()*255.0);
m.vert[vertind].C() = c;
}
return indcl;
}
static void FindStandardWedgeAttributes(WedgeAttribute& wed,const QDomNode nd,const QDomDocument doc)
{
wed.wnsrc = findNodeBySpecificAttributeValue(nd,"input","semantic","NORMAL");
wed.offnm = findStringListAttribute(wed.wn,wed.wnsrc,nd,doc,"NORMAL");
wed.wtsrc = findNodeBySpecificAttributeValue(nd,"input","semantic","TEXCOORD");
if (!wed.wtsrc.isNull())
{
QDomNode src = attributeSourcePerSimplex(nd,doc,"TEXCOORD");
if (isThereTag(src,"accessor"))
{
QDomNodeList wedatts = src.toElement().elementsByTagName("accessor");
wed.stridetx = wedatts.at(0).toElement().attribute("stride").toInt();
}
else
wed.stridetx = 2;
}
//else
// wed.stridetx = 2;
wed.offtx = findStringListAttribute(wed.wt,wed.wtsrc,nd,doc,"TEXCOORD");
wed.wcsrc = findNodeBySpecificAttributeValue(nd,"input","semantic","COLOR");
if (!wed.wcsrc.isNull())
{
QDomNode src = attributeSourcePerSimplex(nd,doc,"COLOR");
if (isThereTag(src,"accessor"))
{
QDomNodeList wedatts = src.toElement().elementsByTagName("accessor");
wed.stridecl = wedatts.at(0).toElement().attribute("stride").toInt();
}
else
wed.stridecl = 3;
}
/*else
wed.stridecl = 3;*/
wed.offcl = findStringListAttribute(wed.wc,wed.wcsrc,nd,doc,"COLOR");
}
static DAEError LoadPolygonalMesh(QDomNodeList& polypatch,ColladaMesh& m,const size_t offset,InfoDAE & info)
{
return E_NOERROR;
}
static DAEError LoadPolygonalListMesh(QDomNodeList& polylist,ColladaMesh& m,const size_t offset,InfoDAE& info,QMap<QString,QString> &materialBinding)
{
if(polylist.isEmpty()) return E_NOERROR;
QDEBUG("****** LoadPolygonalListMesh (initial mesh size %i %i)",m.vert.size(),m.fn);
for(int tript = 0; tript < polylist.size();++tript)
{
QString materialId = polylist.at(tript).toElement().attribute(QString("material"));
QDEBUG("****** material id '%s' -> '%s'",qPrintable(materialId),qPrintable(materialBinding[materialId]));
QString textureFilename;
QDomNode img_node = textureFinder(materialBinding[materialId],textureFilename,*(info.doc));
if(img_node.isNull())
{
QDEBUG("****** but we were not able to find the corresponding image node");
}
int ind_txt = -1;
if (!img_node.isNull())
{
if(info.textureIdMap.contains(textureFilename))
ind_txt=info.textureIdMap[textureFilename];
else
{
QDEBUG("Found use of Texture %s, adding it to texutres",qPrintable(textureFilename));
info.textureIdMap[textureFilename]=m.textures.size();
m.textures.push_back(qPrintable(textureFilename));
ind_txt=info.textureIdMap[textureFilename];
}
}
// number of the attributes associated to each vertex of a face (vert, normal, tex etc)
int faceAttributeNum = polylist.at(tript).toElement().elementsByTagName("input").size();
// the list of indexes composing the size of each polygon.
// The size of this list is the number of the polygons.
QStringList faceSizeList;
valueStringList(faceSizeList,polylist.at(tript),"vcount");
// The long list of indexes composing the various polygons.
// for each polygon there are numvert*numattrib indexes.
QStringList faceIndexList;
valueStringList(faceIndexList,polylist.at(tript),"p");
//int offsetface = (int)m.face.size();
if (faceIndexList.size() != 0 && faceSizeList.size() != 0 )
{
WedgeAttribute wa;
FindStandardWedgeAttributes(wa,polylist.at(tript),*(info.doc));
QDEBUG("******* Start Reading faces. Attributes Offsets: offtx %i - offnm %i - offcl %i",wa.offtx,wa.offnm,wa.offcl);
int faceIndexCnt=0;
int jj = 0;
for(int ff = 0; ff < (int) faceSizeList.size();++ff) // for each polygon
{
int curFaceVertNum = faceSizeList.at(ff).toInt();
MyPolygon<typename ColladaMesh::VertexType> polyTemp(curFaceVertNum);
for(int tt = 0;tt < curFaceVertNum ;++tt) // for each vertex of the polygon
{
int indvt = faceIndexList.at(faceIndexCnt).toInt();
if(faceSizeList.size()<100) QDEBUG("******* Reading face[%3i].V(%i) = %4i (%i-th of the index list) (face has %i vertices)",ff,tt,indvt,faceIndexCnt,curFaceVertNum);
assert(indvt + offset < m.vert.size());
polyTemp._pv[tt] = &(m.vert[indvt + offset]);
faceIndexCnt +=faceAttributeNum;
WedgeTextureAttribute(polyTemp._txc[tt],faceIndexList,ind_txt, wa.wt ,wa.wtsrc, jj + wa.offtx,wa.stridetx);
/****************
if(tri::HasPerWedgeNormal(m)) WedgeNormalAttribute(m,face,wa.wn,wa.wnsrc,ff,jj + wa.offnm,tt);
if(tri::HasPerWedgeColor(m)) WedgeColorAttribute(m,face,wa.wc,wa.wcsrc,ff,jj + wa.offcl,tt);
if(tri::HasPerWedgeTexCoord(m) && ind_txt != -1)
{
WedgeTextureAttribute(m,face,ind_txt,wa.wt,wa.wtsrc,ff,jj + wa.offtx,tt,wa.stride);
}
****************/
jj += faceAttributeNum;
}
AddPolygonToMesh(polyTemp,m);
}
}
}
QDEBUG("****** LoadPolygonalListMesh (final mesh size vn %i vertsize %i - fn %i facesize %i)",m.vn,m.vert.size(),m.fn,m.face.size());
return E_NOERROR;
}
static DAEError AddPolygonToMesh(MyPolygon<typename ColladaMesh::VertexType> &polyTemp, ColladaMesh& m)
{
int vertNum=polyTemp._pv.size();
int triNum= vertNum -2;
typename ColladaMesh::FaceIterator fp=vcg::tri::Allocator<ColladaMesh>::AddFaces(m,triNum);
// Very simple fan triangulation of the polygon.
for(int i=0;i<triNum;++i)
{
assert(fp!=m.face.end());
(*fp).V(0)=polyTemp._pv[0];
(*fp).WT(0)=polyTemp._txc[0];
(*fp).V(1) =polyTemp._pv [i+1];
(*fp).WT(1)=polyTemp._txc[i+1];
(*fp).V(2) =polyTemp._pv[i+2];
(*fp).WT(2)=polyTemp._txc[i+2];
++fp;
}
assert(fp==m.face.end());
return E_NOERROR;
}
static DAEError OldLoadPolygonalListMesh(QDomNodeList& polylist,ColladaMesh& m,const size_t offset,InfoDAE& info)
{
typedef PolygonalMesh< MyPolygon<typename ColladaMesh::VertexType> > PolyMesh;
PolyMesh pm;
//copying vertices
for(typename ColladaMesh::VertexIterator itv = m.vert.begin();itv != m.vert.end();++itv)
{
vcg::Point3f p(itv->P().X(),itv->P().Y(),itv->P().Z());
typename PolyMesh::VertexType v;
v.P() = p;
pm.vert.push_back(v);
}
int polylist_size = polylist.size();
for(int pl = 0; pl < polylist_size;++pl)
{
QString mat = polylist.at(pl).toElement().attribute(QString("material"));
QString textureFilename;
QDomNode txt_node = textureFinder(mat,textureFilename,*(info.doc));
int ind_txt = -1;
if (!txt_node.isNull())
ind_txt = indexTextureByImgNode(*(info.doc),txt_node);
//PolyMesh::PERWEDGEATTRIBUTETYPE att = PolyMesh::NONE;
WedgeAttribute wa;
FindStandardWedgeAttributes(wa,polylist.at(pl),*(info.doc));
QStringList vertcount;
valueStringList(vertcount,polylist.at(pl),"vcount");
int indforpol = findOffSetForASingleSimplex(polylist.at(pl));
int offpols = 0;
int npolig = vertcount.size();
QStringList polyind;
valueStringList(polyind,polylist.at(pl),"p");
for(int ii = 0;ii < npolig;++ii)
{
int nvert = vertcount.at(ii).toInt();
typename PolyMesh::FaceType p(nvert);
for(int iv = 0;iv < nvert;++iv)
{
int index = offset + polyind.at(offpols + iv * indforpol).toInt();
p._pv[iv] = &(pm.vert[index]);
int nmindex = -1;
if (!wa.wnsrc.isNull())
nmindex = offset + polyind.at(offpols + iv * indforpol + wa.offnm).toInt();
int txindex = -1;
if (!wa.wtsrc.isNull())
{
txindex = offset + polyind.at(offpols + iv * indforpol + wa.offtx).toInt();
/*p._txc[iv].U() = wa.wt.at(txindex * 2).toFloat();
p._txc[iv].V() = wa.wt.at(txindex * 2 + 1).toFloat();
p._txc[iv].N() = ind_txt;*/
}
}
pm._pols.push_back(p);
offpols += nvert * indforpol;
}
}
pm.triangulate(m);
return E_NOERROR;
}
/*
Called to load into a given mesh
*/
static DAEError LoadTriangularMesh(QDomNodeList& triNodeList, ColladaMesh& m, const size_t offset, InfoDAE& info,QMap<QString,QString> &materialBinding)
{
if(triNodeList.isEmpty()) return E_NOERROR;
QDEBUG("****** LoadTriangularMesh (initial mesh size %i %i)",m.vn,m.fn);
for(int tript = 0; tript < triNodeList.size();++tript)
{
QString materialId = triNodeList.at(tript).toElement().attribute(QString("material"));
QDEBUG("****** material id '%s' -> '%s'",qPrintable(materialId),qPrintable(materialBinding[materialId]));
QString textureFilename;
QDomNode img_node = textureFinder(materialBinding[materialId],textureFilename,*(info.doc));
if(img_node.isNull())
{
QDEBUG("****** but we were not able to find the corresponding image node");
}
int ind_txt = -1;
if (!img_node.isNull())
{
if(info.textureIdMap.contains(textureFilename))
ind_txt=info.textureIdMap[textureFilename];
else
{
QDEBUG("Found use of Texture %s, adding it to texutres",qPrintable(textureFilename));
info.textureIdMap[textureFilename]=m.textures.size();
m.textures.push_back(qPrintable(textureFilename));
ind_txt=info.textureIdMap[textureFilename];
}
// ind_txt = indexTextureByImgNode(*(info.doc),txt_node);
}
int faceAttributeNum = triNodeList.at(tript).toElement().elementsByTagName("input").size();
QStringList face;
valueStringList(face,triNodeList.at(tript),"p");
int offsetface = (int)m.face.size();
if (face.size() != 0)
{
vcg::tri::Allocator<ColladaMesh>::AddFaces(m,face.size() / (faceAttributeNum * 3));
WedgeAttribute wa;
FindStandardWedgeAttributes(wa,triNodeList.at(tript),*(info.doc));
int jj = 0;
for(int ff = offsetface;ff < (int) m.face.size();++ff)
{
for(unsigned int tt = 0;tt < 3;++tt)
{
int indvt = face.at(jj).toInt();
assert(indvt + offset < m.vert.size());
m.face[ff].V(tt) = &(m.vert[indvt + offset]);
if(tri::HasPerWedgeNormal(m))
WedgeNormalAttribute(m,face,wa.wn,wa.wnsrc,ff,jj + wa.offnm,tt);
if(tri::HasPerVertexColor(m))
{
VertexColorAttribute(m,face,wa.wc,wa.wcsrc,jj + wa.offcl,indvt + offset,wa.stridecl);
}
if(tri::HasPerWedgeTexCoord(m) && ind_txt != -1)
{
WedgeTextureAttribute(m,face,ind_txt,wa.wt,wa.wtsrc,ff,jj + wa.offtx,tt,wa.stridetx);
}
jj += faceAttributeNum;
}
if( ! ( (m.face[ff].V(0) != m.face[ff].V(1)) &&
(m.face[ff].V(0) != m.face[ff].V(2)) &&
(m.face[ff].V(1) != m.face[ff].V(2)) ) )
QDEBUG("********* WARNING face %i, (%i %i %i) is a DEGENERATE FACE!",ff, m.face[ff].V(0) - &m.vert.front(), m.face[ff].V(1) - &m.vert.front(), m.face[ff].V(2) - &m.vert.front());
}
}
}
QDEBUG("****** LoadTriangularMesh (final mesh size %i %i - %i %i)",m.vn,m.vert.size(),m.fn,m.face.size());
return E_NOERROR;
}
static int LoadControllerMesh(ColladaMesh& m, InfoDAE& info, const QDomElement& geo,QMap<QString, QString> materialBindingMap, CallBackPos *cb=0)
{
(void)cb;
assert(geo.tagName() == "controller");
QDomNodeList skinList = geo.toElement().elementsByTagName("skin");
if(skinList.size()!=1) return E_CANTOPEN;
QDomElement skinNode = skinList.at(0).toElement();
QString geomNode_url;
referenceToANodeAttribute(skinNode,"source",geomNode_url);
QDEBUG("Found a controller referencing a skin with url '%s'", qPrintable(geomNode_url));
QDomNode refNode = findNodeBySpecificAttributeValue(*(info.doc),"geometry","id",geomNode_url);
QDomNodeList bindingNodes = skinNode.toElement().elementsByTagName("bind_material");
if( bindingNodes.size()>0) {
QDEBUG("** skin node of a controller has a material binding");
GenerateMaterialBinding(skinNode,materialBindingMap);
}
return LoadGeometry(m, info, refNode.toElement(),materialBindingMap);
}
/* before instancing a geometry you can make a binding that allow you to substitute next material names with other names.
this is very useful for instancing the same geometry with different materials. therefore when you encounter a material name in a mesh, this name can be a 'symbol' that you have to bind.
*/
static bool GenerateMaterialBinding(QDomNode instanceGeomNode, QMap<QString,QString> &binding)
{
QDomNodeList instanceMaterialList=instanceGeomNode.toElement().elementsByTagName("instance_material");
QDEBUG("++++ Found %i instance_material binding",instanceMaterialList.size() );
for(int i=0;i<instanceMaterialList.size();++i)
{
QString symbol = instanceMaterialList.at(i).toElement().attribute("symbol");
QString target = instanceMaterialList.at(i).toElement().attribute("target");
binding[symbol]=target;
QDEBUG("++++++ %s -> %s",qPrintable(symbol),qPrintable(target));
}
return true;
}
/*
Basic function that get in input a node <geometry> with a map from material names to texture names.
this map is necessary because when using a geometry when it is instanced its material can be bind with different names.
if the map fails you should directly search in the material library.
*/
static int LoadGeometry(ColladaMesh& m, InfoDAE& info, const QDomElement& geo, QMap<QString,QString> &materialBinding, CallBackPos *cb=0)
{
assert(geo.tagName() == "geometry");
if (!isThereTag(geo,"mesh")) return E_NOMESH;
if ((cb !=NULL) && (((info.numvert + info.numface)%100)==0) && !(*cb)((100*(info.numvert + info.numface))/(info.numvert + info.numface), "Vertex Loading"))
return E_CANTOPEN;
QDEBUG("**** Loading a Geometry Mesh **** (initial mesh size %i %i)",m.vn,m.fn);
QDomNodeList vertices = geo.toElement().elementsByTagName("vertices");
if (vertices.size() != 1) return E_INCOMPATIBLECOLLADA141FORMAT;
QDomElement vertNode = vertices.at(0).toElement();
QDomNode positionNode = attributeSourcePerSimplex(vertNode,*(info.doc),"POSITION");
if (positionNode.isNull()) return E_NOVERTEXPOSITION;
QStringList geosrcposarr;
valueStringList(geosrcposarr, positionNode, "float_array");
int geosrcposarr_size = geosrcposarr.size();
if ((geosrcposarr_size % 3) != 0)
return E_CANTOPEN;
int nvert = geosrcposarr_size / 3;
size_t offset = m.vert.size();
if (geosrcposarr_size != 0)
{
vcg::tri::Allocator<ColladaMesh>::AddVertices(m,nvert);
QDomNode srcnodenorm = attributeSourcePerSimplex(vertices.at(0),*(info.doc),"NORMAL");
QStringList geosrcvertnorm;
if (!srcnodenorm.isNull())
valueStringList(geosrcvertnorm,srcnodenorm,"float_array");
QDomNode srcnodetext = attributeSourcePerSimplex(vertices.at(0),*(info.doc),"TEXCOORD");
QStringList geosrcverttext;
if (!srcnodetext.isNull())
valueStringList(geosrcverttext,srcnodetext,"float_array");
QDomNode srcnodecolor = attributeSourcePerSimplex(vertices.at(0),*(info.doc),"COLOR");
QDomNodeList accesslist = srcnodecolor.toElement().elementsByTagName("accessor");
QStringList geosrcvertcol;
if (!srcnodecolor.isNull())
valueStringList(geosrcvertcol,srcnodecolor,"float_array");
int ii = 0;
for(size_t vv = offset;vv < m.vert.size();++vv)
{
Point3f positionCoord(geosrcposarr[ii * 3].toFloat(),geosrcposarr[ii * 3 + 1].toFloat(),geosrcposarr[ii * 3 + 2].toFloat());
m.vert[vv].P() = positionCoord;
if (!srcnodenorm.isNull())
{
Point3f normalCoord(geosrcvertnorm[ii * 3].toFloat(),
geosrcvertnorm[ii * 3 + 1].toFloat(),
geosrcvertnorm[ii * 3 + 2].toFloat());
normalCoord.Normalize();
m.vert[vv].N() = normalCoord;
}
if (!srcnodecolor.isNull())
{
if (accesslist.size() > 0)
{
//component per color...obviously we assume they are RGB or RGBA if ARGB you get fancy effects....
if (accesslist.at(0).childNodes().size() == 4)
m.vert[vv].C() = vcg::Color4b(geosrcvertcol[ii * 4].toFloat()*255.0,geosrcvertcol[ii * 4 + 1].toFloat()*255.0,geosrcvertcol[ii * 4 + 2].toFloat()*255.0,geosrcvertcol[ii * 4 + 3].toFloat()*255.0);
else
if (accesslist.at(0).childNodes().size() == 3)
m.vert[vv].C() = vcg::Color4b(geosrcvertcol[ii * 3].toFloat()*255.0,geosrcvertcol[ii * 3 + 1].toFloat()*255.0,geosrcvertcol[ii * 3 + 2].toFloat()*255.0,255.0);
}
}
if (!srcnodetext.isNull())
{
assert((ii * 2 < geosrcverttext.size()) && (ii * 2 + 1 < geosrcverttext.size()));
m.vert[vv].T() = vcg::TexCoord2<float>();
m.vert[vv].T().u() = geosrcverttext[ii * 2].toFloat();
m.vert[vv].T().v() = geosrcverttext[ii * 2 + 1].toFloat();
}
++ii;
}
QDomNodeList tripatch = geo.toElement().elementsByTagName("triangles");
QDomNodeList polypatch = geo.toElement().elementsByTagName("polygons");
QDomNodeList polylist = geo.toElement().elementsByTagName("polylist");
QStringList vertcount;
valueStringList(vertcount,polylist.at(0),"vcount");
int isTri=true;
for (int i=0; i<vertcount.size(); i++)
{
if (vertcount[i]!="3")
{
isTri=false;
break;
}
}
if (isTri && tripatch.isEmpty())
tripatch=polylist;
if (tripatch.isEmpty() && polypatch.isEmpty() && polylist.isEmpty())
return E_NOPOLYGONALMESH;
DAEError err = E_NOERROR;
err = LoadTriangularMesh(tripatch,m,offset,info,materialBinding);
//err = LoadPolygonalMesh(polypatch,m,offset,info);
// err = OldLoadPolygonalListMesh(polylist,m,offset,info);
err = LoadPolygonalListMesh(polylist,m,offset,info,materialBinding);
if (err != E_NOERROR)
return err;
}
QDEBUG("**** Loading a Geometry Mesh **** (final mesh size %i %i - %i %i)",m.vn,m.vert.size(),m.fn,m.face.size());
return E_NOERROR;
}
static void GetTexCoord(const QDomDocument& doc, QStringList &texturefile)
{
QDomNodeList txlst = doc.elementsByTagName("library_images");
for(int img = 0;img < txlst.at(0).childNodes().size();++img)
{
QDomNodeList nlst = txlst.at(0).childNodes().at(img).toElement().elementsByTagName("init_from");
if (nlst.size() > 0)
{
texturefile.push_back( nlst.at(0).firstChild().nodeValue());
}
}
}
// This recursive function add to a mesh the subtree starting from the passed node.
// When you start from a visual_scene, you can find nodes.
// nodes can be directly instanced or referred from the node library.
static void AddNodeToMesh(QDomElement node,
ColladaMesh& m, Matrix44f curTr,
InfoDAE& info)
{
QDEBUG("Starting processing <node> with id %s",qPrintable(node.attribute("id")));
curTr = curTr * getTransfMatrixFromNode(node);
QDomNodeList geomNodeList = node.elementsByTagName("instance_geometry");
for(int ch = 0;ch < geomNodeList.size();++ch)
{
QDomElement instGeomNode= geomNodeList.at(ch).toElement();
if(instGeomNode.parentNode()==node) // process only direct child
{
QDEBUG("** instance_geometry with url %s (intial mesh size %i %i T = %i)",qPrintable(instGeomNode.attribute("url")),m.vn,m.fn,m.textures.size());
//assert(m.textures.size()>0 == HasPerWedgeTexCoord(m));
QString geomNode_url;
referenceToANodeAttribute(instGeomNode,"url",geomNode_url);
QDomNode refNode = findNodeBySpecificAttributeValue(*(info.doc),"geometry","id",geomNode_url);
QDomNodeList bindingNodes = instGeomNode.toElement().elementsByTagName("bind_material");
QMap<QString,QString> materialBindingMap;
if( bindingNodes.size()>0) {
QDEBUG("** instance_geometry has a material binding");
GenerateMaterialBinding(instGeomNode,materialBindingMap);
}
ColladaMesh newMesh;
// newMesh.face.EnableWedgeTex();
LoadGeometry(newMesh, info, refNode.toElement(),materialBindingMap);
tri::UpdatePosition<ColladaMesh>::Matrix(newMesh,curTr);
tri::Append<ColladaMesh,ColladaMesh>::Mesh(m,newMesh);
QDEBUG("** instance_geometry with url %s (final mesh size %i %i - %i %i)",qPrintable(instGeomNode.attribute("url")),m.vn,m.vert.size(),m.fn,m.face.size());
}
}
QDomNodeList controllerNodeList = node.elementsByTagName("instance_controller");
for(int ch = 0;ch < controllerNodeList.size();++ch)
{
QDomElement instContrNode= controllerNodeList.at(ch).toElement();
if(instContrNode.parentNode()==node) // process only direct child
{
QDEBUG("Found a instance_controller with url %s",qPrintable(instContrNode.attribute("url")));
QString controllerNode_url;
referenceToANodeAttribute(instContrNode,"url",controllerNode_url);
QDEBUG("Found a instance_controller with url '%s'", qPrintable(controllerNode_url));
QDomNode refNode = findNodeBySpecificAttributeValue(*(info.doc),"controller","id",controllerNode_url);
QDomNodeList bindingNodes = instContrNode.toElement().elementsByTagName("bind_material");
QMap<QString, QString> materialBindingMap;
if( bindingNodes.size()>0) {
QDEBUG("** instance_controller node of has a material binding");
GenerateMaterialBinding(instContrNode,materialBindingMap);
}
ColladaMesh newMesh;
LoadControllerMesh(newMesh, info, refNode.toElement(),materialBindingMap);
tri::UpdatePosition<ColladaMesh>::Matrix(newMesh,curTr);
tri::Append<ColladaMesh,ColladaMesh>::Mesh(m,newMesh);
}
}
QDomNodeList nodeNodeList = node.elementsByTagName("node");
for(int ch = 0;ch < nodeNodeList.size();++ch)
{
if(nodeNodeList.at(ch).parentNode()==node) // process only direct child
AddNodeToMesh(nodeNodeList.at(ch).toElement(), m,curTr, info);
}
QDomNodeList instanceNodeList = node.elementsByTagName("instance_node");
for(int ch = 0;ch < instanceNodeList.size();++ch)
{
if(instanceNodeList.at(ch).parentNode()==node) // process only direct child
{
QDomElement instanceNode = instanceNodeList.at(ch).toElement();
QString node_url;
referenceToANodeAttribute(instanceNode,"url",node_url);
QDEBUG("Found a instance_node with url '%s'", qPrintable(node_url));
QDomNode refNode = findNodeBySpecificAttributeValue(*(info.doc),"node","id",node_url);
if(refNode.isNull())
QDEBUG("findNodeBySpecificAttributeValue returned a null node for %s",qPrintable(node_url));
AddNodeToMesh(refNode.toElement(), m,curTr, info);
}
}
}
// Retrieve the transformation matrix that is defined in the childs of a node.
// used during the recursive descent.
static Matrix44f getTransfMatrixFromNode(const QDomElement parentNode)
{
QDEBUG("getTrans form node with tag %s",qPrintable(parentNode.tagName()));
assert(parentNode.tagName() == "node");
std::vector<QDomNode> rotationList;
QDomNode matrixNode;
QDomNode translationNode;
for(int ch = 0;ch < parentNode.childNodes().size();++ch)
{
if (parentNode.childNodes().at(ch).nodeName() == "rotate")
rotationList.push_back(parentNode.childNodes().at(ch));
if (parentNode.childNodes().at(ch).nodeName() == "translate")
translationNode = parentNode.childNodes().at(ch);
if (parentNode.childNodes().at(ch).nodeName() == "matrix")
matrixNode = parentNode.childNodes().at(ch);
}
Matrix44f rotM; rotM.SetIdentity();
Matrix44f transM; transM.SetIdentity();
if (!translationNode.isNull()) ParseTranslation(transM,translationNode);
if (!rotationList.empty()) ParseRotationMatrix(rotM,rotationList);
if (!matrixNode.isNull())
{
ParseMatrixNode(transM,matrixNode);
return transM;
}
return transM*rotM;
}
public:
//merge all meshes in the collada's file in the templeted mesh m
//I assume the mesh
static int Open(OpenMeshType& m,const char* filename, InfoDAE& info, CallBackPos *cb=0)
{
(void)cb;
QDEBUG("----- Starting the processing of %s ------",filename);
//AdditionalInfoDAE& inf = new AdditionalInfoDAE();
//info = new InfoDAE();
QDomDocument* doc = new QDomDocument(filename);
info.doc = doc;
QFile file(filename);
if (!file.open(QIODevice::ReadOnly))
return E_CANTOPEN;
if (!doc->setContent(&file))
{
file.close();
return E_CANTOPEN;
}
file.close();
//GetTexture(*(info.doc),inf);
// GenerateMaterialToTextureMap(info);
//scene->instance_visual_scene
QDomNodeList scenes = info.doc->elementsByTagName("scene");
int scn_size = scenes.size();
if (scn_size == 0)
return E_NO3DSCENE;
QDEBUG("File Contains %i Scenes",scenes.size());
int problem = E_NOERROR;
//bool found_a_mesh = false;
//Is there geometry in the file?
//bool geoinst_found = false;
// The main loading loop
// for each scene in COLLADA FILE
/*
Some notes on collada structure.
top level nodes are :
<asset>
<library_images>
<library_materials>
<library_effects>
<library_geometries>
<library_visual_scene>
<scene>
The REAL top root is the <scene> that can contains one of more (instance of) <visual_scene>.
<visual_scene> can be directly written there (check!) or instanced from their definition in the <library_visual_scene>
each <visual_scene> contains a hierarchy of <node>
each <node> contains
transformation
other nodes (to build up a hierarchy)
instance of geometry
instance of controller
instance can be direct or refers name of stuff described in a library.
An instance of geometry node should contain the <mesh> node and as a son of the <instance geometry> the material node (again referenced from a library)
-- structure of the geometry node --
*/
for(int scn = 0;scn < scn_size;++scn)
{
QDomNodeList instscenes = scenes.at(scn).toElement().elementsByTagName("instance_visual_scene");
int instscn_size = instscenes.size();
QDEBUG("Scene %i contains %i instance_visual_scene ",scn,instscn_size);
if (instscn_size == 0) return E_INCOMPATIBLECOLLADA141FORMAT;
//for each scene instance in a COLLADA scene
for(int instscn = 0;instscn < instscn_size; ++instscn)
{
QString libscn_url;
referenceToANodeAttribute(instscenes.at(instscn),"url",libscn_url);
QDEBUG("instance_visual_scene %i refers %s ",instscn,qPrintable(libscn_url));
// QDomNode nd = QDomNode(*(inf->doc));
QDomNode visscn = findNodeBySpecificAttributeValue(*(info.doc),"visual_scene","id",libscn_url);
if(visscn.isNull()) return E_UNREFERENCEBLEDCOLLADAATTRIBUTE;
//assert (visscn.toElement().Attribute("id") == libscn_url);
//for each node in the libscn_url visual scene
QDomNodeList visscn_child = visscn.childNodes();
QDEBUG("instance_visual_scene %s has %i children",qPrintable(libscn_url),visscn_child.size());
// for each direct child of a visual scene process it
for(int chdind = 0; chdind < visscn_child.size();++chdind)
{
QDomElement node=visscn_child.at(chdind).toElement();
if(node.isNull()) continue;
QDEBUG("Processing Visual Scene child %i - of type '%s'",chdind,qPrintable(node.tagName()));
Matrix44f baseTr; baseTr.SetIdentity();
if(node.toElement().tagName()=="node")
{
ColladaMesh newMesh;
AddNodeToMesh(node.toElement(), newMesh, baseTr,info);
tri::Append<OpenMeshType,ColladaMesh>::Mesh(m,newMesh);
}
} // end for each node of a given scene
} // end for each visual scene instance
} // end for each scene instance
return problem;
}
static bool LoadMask(const char * filename, InfoDAE& info)
{
bool bHasPerWedgeTexCoord = false;
bool bHasPerWedgeNormal = false;
//bool bHasPerWedgeColor = false;
bool bHasPerVertexColor = false;
bool bHasPerFaceColor = false;
bool bHasPerVertexNormal = false;
bool bHasPerVertexText = false;
QDomDocument* doc = new QDomDocument(filename);
QFile file(filename);
if (!file.open(QIODevice::ReadOnly))
return false;
if (!doc->setContent(&file))
{
file.close();
return false;
}
file.close();
QStringList textureFileList;
info.doc = doc;
GetTexCoord(*(info.doc),textureFileList);
QDomNodeList scenes = info.doc->elementsByTagName("scene");
int scn_size = scenes.size();
//Is there geometry in the file?
bool geoinst_found = false;
//for each scene in COLLADA FILE
for(int scn = 0;scn < scn_size;++scn)
{
QDomNodeList instscenes = scenes.at(scn).toElement().elementsByTagName("instance_visual_scene");
int instscn_size = instscenes.size();
if (instscn_size == 0) return false;
//for each scene instance in a COLLADA scene
for(int instscn = 0;instscn < instscn_size; ++instscn)
{
QString libscn_url;
referenceToANodeAttribute(instscenes.at(instscn),"url",libscn_url);
QDomNode nd = QDomNode(*(info.doc));
QDomNode visscn = findNodeBySpecificAttributeValue(*(info.doc),"visual_scene","id",libscn_url);
if(visscn.isNull()) return false;
//for each node in the libscn_url visual scene
//QDomNodeList& visscn_child = visscn.childNodes();
QDomNodeList visscn_child = visscn.childNodes();
//for each direct child of a libscn_url visual scene find if there is some geometry instance
for(int chdind = 0; chdind < visscn_child.size();++chdind)
{
//QDomNodeList& geoinst = visscn_child.at(chdind).toElement().elementsByTagName("instance_geometry");
QDomNodeList geoinst = visscn_child.at(chdind).toElement().elementsByTagName("instance_geometry");
int geoinst_size = geoinst.size();
if (geoinst_size != 0)
{
geoinst_found |= true;
QDomNodeList geolib = info.doc->elementsByTagName("library_geometries");
//assert(geolib.size() == 1);
if (geolib.size() != 1)
return false;
//!!!!!!!!!!!!!!!!!here will be the code for geometry transformations!!!!!!!!!!!!!!!!!!!!!!
info.numvert = 0;
info.numface = 0;
for(int geoinst_ind = 0;geoinst_ind < geoinst_size;++geoinst_ind)
{
QString geo_url;
referenceToANodeAttribute(geoinst.at(geoinst_ind),"url",geo_url);
QDomNode geo = findNodeBySpecificAttributeValue(geolib.at(0),"geometry","id",geo_url);
if (geo.isNull())
return false;
QDomNodeList vertlist = geo.toElement().elementsByTagName("vertices");
for(int vert = 0;vert < vertlist.size();++vert)
{
QDomNode no;
no = findNodeBySpecificAttributeValue(vertlist.at(vert),"input","semantic","POSITION");
QString srcurl;
referenceToANodeAttribute(no,"source",srcurl);
no = findNodeBySpecificAttributeValue(geo,"source","id",srcurl);
QDomNodeList fa = no.toElement().elementsByTagName("float_array");
assert(fa.size() == 1);
info.numvert += (fa.at(0).toElement().attribute("count").toInt() / 3);
no = findNodeBySpecificAttributeValue(vertlist.at(vert),"input","semantic","COLOR");
if (!no.isNull())
bHasPerVertexColor = true;
no = findNodeBySpecificAttributeValue(vertlist.at(vert),"input","semantic","NORMAL");
if (!no.isNull())
bHasPerVertexNormal = true;
no = findNodeBySpecificAttributeValue(vertlist.at(vert),"input","semantic","TEXCOORD");
if (!no.isNull())
bHasPerVertexText = true;
}
const char* arr[] = {"triangles","polylist","polygons"};
for(unsigned int tt= 0;tt < 3;++tt)
{
QDomNodeList facelist = geo.toElement().elementsByTagName(arr[tt]);
for(int face = 0;face < facelist.size();++face)
{
info.numface += facelist.at(face).toElement().attribute("count").toInt() ;
QDomNode no;
no = findNodeBySpecificAttributeValue(facelist.at(face),"input","semantic","NORMAL");
if (!no.isNull())
bHasPerWedgeNormal = true;
no = findNodeBySpecificAttributeValue(facelist.at(face),"input","semantic","COLOR");
if (!no.isNull())
bHasPerVertexColor = true;
no = findNodeBySpecificAttributeValue(facelist.at(face),"input","semantic","TEXCOORD");
if (!no.isNull())
bHasPerWedgeTexCoord = true;
}
}
}
}
}
}
}
if (!geoinst_found)
{
QDomNodeList geolib = info.doc->elementsByTagName("library_geometries");
//assert(geolib.size() == 1);
if (geolib.size() != 1)
return false;
QDomNodeList geochild = geolib.at(0).toElement().elementsByTagName("geometry");
//!!!!!!!!!!!!!!!!!here will be the code for geometry transformations!!!!!!!!!!!!!!!!!!!!!!
info.numvert = 0;
info.numface = 0;
for(int geoinst_ind = 0;geoinst_ind < geochild.size();++geoinst_ind)
{
QDomNodeList vertlist = geochild.at(geoinst_ind).toElement().elementsByTagName("vertices");
for(int vert = 0;vert < vertlist.size();++vert)
{
QDomNode no;
no = findNodeBySpecificAttributeValue(vertlist.at(vert),"input","semantic","POSITION");
QString srcurl;
referenceToANodeAttribute(no,"source",srcurl);
no = findNodeBySpecificAttributeValue(geochild.at(geoinst_ind),"source","id",srcurl);
QDomNodeList fa = no.toElement().elementsByTagName("float_array");
assert(fa.size() == 1);
info.numvert += (fa.at(0).toElement().attribute("count").toInt() / 3);
no = findNodeBySpecificAttributeValue(vertlist.at(vert),"input","semantic","COLOR");
if (!no.isNull())
bHasPerVertexColor = true;
no = findNodeBySpecificAttributeValue(vertlist.at(vert),"input","semantic","NORMAL");
if (!no.isNull())
bHasPerVertexNormal = true;
no = findNodeBySpecificAttributeValue(vertlist.at(vert),"input","semantic","TEXCOORD");
if (!no.isNull())
bHasPerVertexText = true;
}
QDomNodeList facelist = geochild.at(geoinst_ind).toElement().elementsByTagName("triangles");
for(int face = 0;face < facelist.size();++face)
{
info.numface += facelist.at(face).toElement().attribute("count").toInt() ;
QDomNode no;
no = findNodeBySpecificAttributeValue(facelist.at(face),"input","semantic","NORMAL");
if (!no.isNull())
bHasPerWedgeNormal = true;
no = findNodeBySpecificAttributeValue(facelist.at(face),"input","semantic","TEXCOORD");
if (!no.isNull())
bHasPerWedgeTexCoord = true;
}
}
}
info.mask = 0;
if (bHasPerWedgeTexCoord)
info.mask |= vcg::tri::io::Mask::IOM_WEDGTEXCOORD;
if (bHasPerWedgeNormal)
info.mask |= vcg::tri::io::Mask::IOM_WEDGNORMAL;
if (bHasPerVertexColor)
info.mask |= vcg::tri::io::Mask::IOM_VERTCOLOR;
if (bHasPerFaceColor)
info.mask |= vcg::tri::io::Mask::IOM_FACECOLOR;
if (bHasPerVertexNormal)
info.mask |= vcg::tri::io::Mask::IOM_VERTNORMAL;
if (bHasPerVertexText)
info.mask |= vcg::tri::io::Mask::IOM_VERTTEXCOORD;
delete (info.doc);
info.doc = NULL;
//addinfo = info;
return true;
}
};
}
}
}
#endif
| {'content_hash': 'b46318ba14966e180200c6a8de356698', 'timestamp': '', 'source': 'github', 'line_count': 1031, 'max_line_length': 214, 'avg_line_length': 39.19592628516004, 'alnum_prop': 0.628665462374106, 'repo_name': 'hlzz/dotfiles', 'id': '05950e794e95f27aba2078d2b64ea0a0af4e3065', 'size': '42129', 'binary': False, 'copies': '2', 'ref': 'refs/heads/master', 'path': 'graphics/vcglib/wrap/io_trimesh/import_dae.h', 'mode': '33188', 'license': 'bsd-3-clause', 'language': [{'name': 'AppleScript', 'bytes': '1240'}, {'name': 'Arc', 'bytes': '38'}, {'name': 'Assembly', 'bytes': '449468'}, {'name': 'Batchfile', 'bytes': '16152'}, {'name': 'C', 'bytes': '102303195'}, {'name': 'C++', 'bytes': '155056606'}, {'name': 'CMake', 'bytes': '7200627'}, {'name': 'CSS', 'bytes': '179330'}, {'name': 'Cuda', 'bytes': '30026'}, {'name': 'D', 'bytes': '2152'}, {'name': 'Emacs Lisp', 'bytes': '14892'}, {'name': 'FORTRAN', 'bytes': '5276'}, {'name': 'Forth', 'bytes': '3637'}, {'name': 'GAP', 'bytes': '14495'}, {'name': 'GLSL', 'bytes': '438205'}, {'name': 'Gnuplot', 'bytes': '327'}, {'name': 'Groff', 'bytes': '518260'}, {'name': 'HLSL', 'bytes': '965'}, {'name': 'HTML', 'bytes': '2003175'}, {'name': 'Haskell', 'bytes': '10370'}, {'name': 'IDL', 'bytes': '2466'}, {'name': 'Java', 'bytes': '219109'}, {'name': 'JavaScript', 'bytes': '1618007'}, {'name': 'Lex', 'bytes': '119058'}, {'name': 'Lua', 'bytes': '23167'}, {'name': 'M', 'bytes': '1080'}, {'name': 'M4', 'bytes': '292475'}, {'name': 'Makefile', 'bytes': '7112810'}, {'name': 'Matlab', 'bytes': '1582'}, {'name': 'NSIS', 'bytes': '34176'}, {'name': 'Objective-C', 'bytes': '65312'}, {'name': 'Objective-C++', 'bytes': '269995'}, {'name': 'PAWN', 'bytes': '4107117'}, {'name': 'PHP', 'bytes': '2690'}, {'name': 'Pascal', 'bytes': '5054'}, {'name': 'Perl', 'bytes': '485508'}, {'name': 'Pike', 'bytes': '1338'}, {'name': 'Prolog', 'bytes': '5284'}, {'name': 'Python', 'bytes': '16799659'}, {'name': 'QMake', 'bytes': '89858'}, {'name': 'Rebol', 'bytes': '291'}, {'name': 'Ruby', 'bytes': '21590'}, {'name': 'Scilab', 'bytes': '120244'}, {'name': 'Shell', 'bytes': '2266191'}, {'name': 'Slash', 'bytes': '1536'}, {'name': 'Smarty', 'bytes': '1368'}, {'name': 'Swift', 'bytes': '331'}, {'name': 'Tcl', 'bytes': '1911873'}, {'name': 'TeX', 'bytes': '11981'}, {'name': 'Verilog', 'bytes': '3893'}, {'name': 'VimL', 'bytes': '595114'}, {'name': 'XSLT', 'bytes': '62675'}, {'name': 'Yacc', 'bytes': '307000'}, {'name': 'eC', 'bytes': '366863'}]} |
package xitrum.routing
private object IgnoredPackages {
/**
* @param relPath "/" separated path to the .class file that may contain
* Xitrum routes
*
* @return true for those (java/..., javax/... etc.) that should be ignored
* because they obviously don't contain Xitrum routes, to speed up the
* scanning of routes
*/
def isIgnored(relPath: String): Boolean = {
// Standard Java and Scala
relPath.startsWith("java/") ||
relPath.startsWith("javafx/") ||
relPath.startsWith("javax/") ||
relPath.startsWith("scala/") ||
relPath.startsWith("sun/") ||
relPath.startsWith("com/sun/") ||
// Others
relPath.startsWith("akka/") ||
relPath.startsWith("ch/qos/logback") ||
relPath.startsWith("com/beachape/filemanagement") ||
relPath.startsWith("com/codahale/metrics") ||
relPath.startsWith("com/esotericsoftware/kryo/") ||
relPath.startsWith("com/esotericsoftware/reflectasm/") ||
relPath.startsWith("com/fasterxml/jackson/") ||
relPath.startsWith("com/google/") ||
relPath.startsWith("com/thoughtworks/paranamer/") ||
relPath.startsWith("com/twitter/") ||
relPath.startsWith("com/typesafe/") ||
relPath.startsWith("glokka/") ||
relPath.startsWith("io/netty/") ||
relPath.startsWith("javassist/") ||
relPath.startsWith("nl/grons/metrics/") ||
relPath.startsWith("org/aopalliance/") ||
relPath.startsWith("org/apache/") ||
relPath.startsWith("org/codehaus/commons/") ||
relPath.startsWith("org/codehaus/janino/") ||
relPath.startsWith("org/cyberneko/html/") ||
relPath.startsWith("org/fusesource/hawtjni/") ||
relPath.startsWith("org/fusesource/leveldbjni/") ||
relPath.startsWith("org/fusesource/scalamd/") ||
relPath.startsWith("org/fusesource/scalate/") ||
relPath.startsWith("org/jboss/") ||
relPath.startsWith("org/json4s/") ||
relPath.startsWith("org/iq80/leveldb") ||
relPath.startsWith("org/mozilla/") ||
relPath.startsWith("org/objectweb/asm/") ||
relPath.startsWith("org/objenesis/") ||
relPath.startsWith("org/openid4java/") ||
relPath.startsWith("org/slf4j/") ||
relPath.startsWith("org/slf4s/") ||
relPath.startsWith("org/w3c/") ||
relPath.startsWith("org/xml/") ||
relPath.startsWith("org/uncommons/maths/") ||
relPath.startsWith("rx/")
}
}
| {'content_hash': 'ebf29b1ec241e52b4f255c43c8051a34', 'timestamp': '', 'source': 'github', 'line_count': 59, 'max_line_length': 77, 'avg_line_length': 39.932203389830505, 'alnum_prop': 0.6608658743633277, 'repo_name': 'xitrum-framework/xitrum', 'id': '43cc3ed904aa475c0daad1c5ef9737b2c8d3e22c', 'size': '2356', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/main/scala/xitrum/routing/IgnoredPackages.scala', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '1159'}, {'name': 'JavaScript', 'bytes': '21940'}, {'name': 'Scala', 'bytes': '492881'}]} |
<div class="problem-number">Problem 265</div>
<div class="problem-name">Binary Circles</div>
<div class="problem-content">
<p>2<sup>N</sup> binary digits can be placed in a circle so that all the N-digit clockwise subsequences are distinct.</p>
<p>For N=3, two such circular arrangements are possible, ignoring rotations:</p>
<div align="center"><img src="/files/p_265_BinaryCircles.gif"></div>
<p>For the first arrangement, the 3-digit subsequences, in clockwise order, are:<br> 000, 001, 010, 101, 011, 111, 110 and 100.</p>
<p>Each circular arrangement can be encoded as a number by concatenating
the binary digits starting with the subsequence of all zeros as the
most significant bits and proceeding clockwise. The two arrangements for
N=3 are thus represented as 23 and 29:
</p>
<div align="center">00010111 <sub>2</sub> = 23</div>
<div align="center">00011101 <sub>2</sub> = 29</div>
<p>Calling S(N) the sum of the unique numeric representations, we can see that S(3) = 23 + 29 = 52.</p>
<p>Find S(5).</p>
</div>
| {'content_hash': '597b959a938e3a704db471171701ecc0', 'timestamp': '', 'source': 'github', 'line_count': 26, 'max_line_length': 131, 'avg_line_length': 39.69230769230769, 'alnum_prop': 0.7199612403100775, 'repo_name': 'briankung/sprint', 'id': '30973b53ab2b554d2f41c3cddeda14209b896452', 'size': '1036', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'app/views/problems/all_problems/_p_265.html', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '4905'}, {'name': 'CoffeeScript', 'bytes': '844'}, {'name': 'Gherkin', 'bytes': '3141'}, {'name': 'HTML', 'bytes': '675241'}, {'name': 'JavaScript', 'bytes': '2325'}, {'name': 'Ruby', 'bytes': '100151'}]} |
namespace ggk {
struct Mgmt
{
//
// Constants
//
// The length of the controller's name (not including null terminator)
static const int kMaxAdvertisingNameLength = 248;
// The length of the controller's short name (not including null terminator)
static const int kMaxAdvertisingShortNameLength = 10;
//
// Types
//
// These indices should match those in HciAdapter::kEventTypeNames
enum EventTypes
{
EInvalidEvent = 0x0000,
ECommandCompleteEvent = 0x0001,
ECommandStatusEvent = 0x0002,
EControllerErrorEvent = 0x0003,
EIndexAddedEvent = 0x0004,
EIndexRemovedEvent = 0x0005,
ENewSettingsEvent = 0x0006,
EClassOfDeviceChangedEvent = 0x0007,
ELocalNameChangedEvent = 0x0008,
ENewLinkKeyEvent = 0x0009,
ENewLongTermKeyEvent = 0x000A,
EDeviceConnectedEvent = 0x000B,
EDeviceDisconnectedEvent = 0x000C,
EConnectFailedEvent = 0x000D,
EPINCodeRequestEvent = 0x000E,
EUserConfirmationRequestEvent = 0x000F,
EUserPasskeyRequestEvent = 0x0010,
EAuthenticationFailedEvent = 0x0011,
EDeviceFoundEvent = 0x0012,
EDiscoveringEvent = 0x0013,
EDeviceBlockedEvent = 0x0014,
EDeviceUnblockedEvent = 0x0015,
EDeviceUnpairedEvent = 0x0016,
EPasskeyNotifyEvent = 0x0017,
ENewIdentityResolvingKeyEvent = 0x0018,
ENewSignatureResolvingKeyEvent = 0x0019,
EDeviceAddedEvent = 0x001a,
EDeviceRemovedEvent = 0x001b,
ENewConnectionParameterEvent = 0x001c,
EUnconfiguredIndexAddedEvent = 0x001d,
EUnconfiguredIndexRemovedEvent = 0x001e,
ENewConfigurationOptionsEvent = 0x001f,
EExtendedIndexAddedEvent = 0x0020,
EExtendedIndexRemovedEvent = 0x0021,
ELocalOutOfBandExtendedDataUpdatedEvent = 0x0022,
EAdvertisingAddedEvent = 0x0023,
EAdvertisingRemovedEvent = 0x0024,
EExtendedControllerInformationChangedEvent = 0x0025
};
// These indices should match those in HciAdapter::kCommandCodeNames
enum CommandCodes
{
EInvalidCommand = 0x0000,
EReadVersionInformationCommand = 0x0001,
EReadSupportedCommandsCommand = 0x0002,
EReadControllerIndexListCommand = 0x0003,
EReadControllerInformationCommand = 0x0004,
ESetPoweredCommand = 0x0005,
ESetDiscoverableCommand = 0x0006,
ESetConnectableCommand = 0x0007,
ESetFastConnectableCommand = 0x0008,
ESetBondableCommand = 0x0009,
ESetLinkSecurityCommand = 0x000A,
ESetSecureSimplePairingCommand = 0x000B,
ESetHighSpeedCommand = 0x000C,
ESetLowEnergyCommand = 0x000D,
ESetDeviceClass = 0x000E,
ESetLocalNameCommand = 0x000F,
EAddUUIDCommand = 0x0010,
ERemoveUUIDCommand = 0x0011,
ELoadLinkKeysCommand = 0x0012,
ELoadLongTermKeysCommand = 0x0013,
EDisconnectCommand = 0x0014,
EGetConnectionsCommand = 0x0015,
EPINCodeReplyCommand = 0x0016,
EPINCodeNegativeReplyCommand = 0x0017,
ESetIOCapabilityCommand = 0x0018,
EPairDeviceCommand = 0x0019,
ECancelPairDeviceCommand = 0x001A,
EUnpairDeviceCommand = 0x001B,
EUserConfirmationReplyCommand = 0x001C,
EUserConfirmationNegativeReplyCommand = 0x001D,
EUserPasskeyReplyCommand = 0x001E,
EUserPasskeyNegativeReplyCommand = 0x001F,
EReadLocalOutOfBandDataCommand = 0x0020,
EAddRemoteOutOfBandDataCommand = 0x0021,
ERemoveRemoteOutOfBandDataCommand = 0x0022,
EStartDiscoveryCommand = 0x0023,
EStopDiscoveryCommand = 0x0024,
EConfirmNameCommand = 0x0025,
EBlockDeviceCommand = 0x0026,
EUnblockDeviceCommand = 0x0027,
ESetDeviceIDCommand = 0x0028,
ESetAdvertisingCommand = 0x0029,
ESetBREDRCommand = 0x002A,
ESetStaticAddressCommand = 0x002B,
ESetScanParametersCommand = 0x002C,
ESetSecureConnectionsCommand = 0x002D,
ESetDebugKeysCommand = 0x002E,
ESetPrivacyCommand = 0x002F,
ELoadIdentityResolvingKeysCommand = 0x0030,
EGetConnectionInformationCommand = 0x0031,
EGetClockInformationCommand = 0x0032,
EAddDeviceCommand = 0x0033,
ERemoveDeviceCommand = 0x0034,
ELoadConnectionParametersCommand = 0x0035,
EReadUnconfiguredControllerIndexListCommand = 0x0036,
EReadControllerConfigurationInformationCommand = 0x0037,
ESetExternalConfigurationCommand = 0x0038,
ESetPublicAddressCommand = 0x0039,
EStartServiceDiscoveryCommand = 0x003a,
EReadLocalOutOfBandExtendedDataCommand = 0x003b,
EReadExtendedControllerIndexListCommand = 0x003c,
EReadAdvertisingFeaturesCommand = 0x003d,
EAddAdvertisingCommand = 0x003e,
ERemoveAdvertisingCommand = 0x003f,
EGetAdvertisingSizeInformationCommand = 0x0040,
EStartLimitedDiscoveryCommand = 0x0041,
EReadExtendedControllerInformationCommand = 0x0042,
ESetAppearanceCommand = 0x0043
};
// Construct the Mgmt device
//
// Set `controllerIndex` to the zero-based index of the device as recognized by the OS. If this parameter is omitted, the index
// of the first device (0) will be used.
Mgmt(uint16_t controllerIndex = kDefaultControllerIndex);
// Set the adapter name and short name
//
// The inputs `name` and `shortName` may be truncated prior to setting them on the adapter. To ensure that `name` and
// `shortName` conform to length specifications prior to calling this method, see the constants `kMaxAdvertisingNameLength` and
// `kMaxAdvertisingShortNameLength`. In addition, the static methods `truncateName()` and `truncateShortName()` may be helpful.
//
// Returns true on success, otherwise false
bool setName(std::string name, std::string shortName);
// Sets discoverable mode
// 0x00 disables discoverable
// 0x01 enables general discoverable
// 0x02 enables limited discoverable
// Timeout is the time in seconds. For 0x02, the timeout value is required.
bool setDiscoverable(uint8_t disc, uint16_t timeout);
// Set a setting state to 'newState'
//
// Many settings are set the same way, this is just a convenience routine to handle them all
//
// Returns true on success, otherwise false
bool setState(uint16_t commandCode, uint16_t controllerId, uint8_t newState);
// Set the powered state to `newState` (true = powered on, false = powered off)
//
// Returns true on success, otherwise false
bool setPowered(bool newState);
// Set the BR/EDR state to `newState` (true = enabled, false = disabled)
//
// Returns true on success, otherwise false
bool setBredr(bool newState);
// Set the Secure Connection state (0 = disabled, 1 = enabled, 2 = secure connections only mode)
//
// Returns true on success, otherwise false
bool setSecureConnections(uint8_t newState);
// Set the bondable state to `newState` (true = enabled, false = disabled)
//
// Returns true on success, otherwise false
bool setBondable(bool newState);
// Set the connectable state to `newState` (true = enabled, false = disabled)
//
// Returns true on success, otherwise false
bool setConnectable(bool newState);
// Set the LE state to `newState` (true = enabled, false = disabled)
//
// Returns true on success, otherwise false
bool setLE(bool newState);
// Set the advertising state to `newState` (0 = disabled, 1 = enabled (with consideration towards the connectable setting),
// 2 = enabled in connectable mode).
//
// Returns true on success, otherwise false
bool setAdvertising(uint8_t newState);
//
// Utilitarian
//
// Truncates the string `name` to the maximum allowed length for an adapter name. If `name` needs no truncation, a copy of
// `name` is returned.
static std::string truncateName(const std::string &name);
// Truncates the string `name` to the maximum allowed length for an adapter short-name. If `name` needs no truncation, a copy
// of `name` is returned.
static std::string truncateShortName(const std::string &name);
private:
//
// Data members
//
// The default controller index (the first device)
uint16_t controllerIndex;
// Default controller index
static const uint16_t kDefaultControllerIndex = 0;
};
}; // namespace ggk
| {'content_hash': 'b93827ba26a62c97d1ff51cfffbadbe4', 'timestamp': '', 'source': 'github', 'line_count': 225, 'max_line_length': 128, 'avg_line_length': 47.78666666666667, 'alnum_prop': 0.5562686011904762, 'repo_name': 'nettlep/gobbledegook', 'id': 'b76fa5ced46cf29d3b8e4513d1a900ba9280474a', 'size': '11833', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/Mgmt.h', 'mode': '33188', 'license': 'bsd-3-clause', 'language': [{'name': 'C', 'bytes': '13389'}, {'name': 'C++', 'bytes': '342592'}, {'name': 'M4', 'bytes': '21421'}, {'name': 'Makefile', 'bytes': '84664'}, {'name': 'Shell', 'bytes': '58752'}]} |
using namespace std;
class CAddrManTest : public CAddrMan
{
uint64_t state;
public:
CAddrManTest()
{
state = 1;
}
//! Ensure that bucket placement is always the same for testing purposes.
void MakeDeterministic()
{
nKey.SetNull();
seed_insecure_rand(true);
}
int RandomInt(int nMax)
{
state = (CHashWriter(SER_GETHASH, 0) << state).GetHash().GetCheapHash();
return (unsigned int)(state % nMax);
}
CAddrInfo* Find(const CNetAddr& addr, int* pnId = NULL)
{
return CAddrMan::Find(addr, pnId);
}
CAddrInfo* Create(const CAddress& addr, const CNetAddr& addrSource, int* pnId = NULL)
{
return CAddrMan::Create(addr, addrSource, pnId);
}
void Delete(int nId)
{
CAddrMan::Delete(nId);
}
};
static CNetAddr ResolveIP(const char* ip)
{
CNetAddr addr;
BOOST_CHECK_MESSAGE(LookupHost(ip, addr, false), strprintf("failed to resolve: %s", ip));
return addr;
}
static CNetAddr ResolveIP(std::string ip)
{
return ResolveIP(ip.c_str());
}
static CService ResolveService(const char* ip, int port = 0)
{
CService serv;
BOOST_CHECK_MESSAGE(Lookup(ip, serv, port, false), strprintf("failed to resolve: %s:%i", ip, port));
return serv;
}
static CService ResolveService(std::string ip, int port = 0)
{
return ResolveService(ip.c_str(), port);
}
BOOST_FIXTURE_TEST_SUITE(addrman_tests, BasicTestingSetup)
BOOST_AUTO_TEST_CASE(addrman_simple)
{
CAddrManTest addrman;
// Set addrman addr placement to be deterministic.
addrman.MakeDeterministic();
CNetAddr source = ResolveIP("252.2.2.2");
// Test 1: Does Addrman respond correctly when empty.
BOOST_CHECK(addrman.size() == 0);
CAddrInfo addr_null = addrman.Select();
BOOST_CHECK(addr_null.ToString() == "[::]:0");
// Test 2: Does Addrman::Add work as expected.
CService addr1 = ResolveService("250.1.1.1", 8333);
addrman.Add(CAddress(addr1, NODE_NONE), source);
BOOST_CHECK(addrman.size() == 1);
CAddrInfo addr_ret1 = addrman.Select();
BOOST_CHECK(addr_ret1.ToString() == "250.1.1.1:8333");
// Test 3: Does IP address deduplication work correctly.
// Expected dup IP should not be added.
CService addr1_dup = ResolveService("250.1.1.1", 8333);
addrman.Add(CAddress(addr1_dup, NODE_NONE), source);
BOOST_CHECK(addrman.size() == 1);
// Test 5: New table has one addr and we add a diff addr we should
// have two addrs.
CService addr2 = ResolveService("250.1.1.2", 8333);
addrman.Add(CAddress(addr2, NODE_NONE), source);
BOOST_CHECK(addrman.size() == 2);
// Test 6: AddrMan::Clear() should empty the new table.
addrman.Clear();
BOOST_CHECK(addrman.size() == 0);
CAddrInfo addr_null2 = addrman.Select();
BOOST_CHECK(addr_null2.ToString() == "[::]:0");
}
BOOST_AUTO_TEST_CASE(addrman_ports)
{
CAddrManTest addrman;
// Set addrman addr placement to be deterministic.
addrman.MakeDeterministic();
CNetAddr source = ResolveIP("252.2.2.2");
BOOST_CHECK(addrman.size() == 0);
// Test 7; Addr with same IP but diff port does not replace existing addr.
CService addr1 = ResolveService("250.1.1.1", 8333);
addrman.Add(CAddress(addr1, NODE_NONE), source);
BOOST_CHECK(addrman.size() == 1);
CService addr1_port = ResolveService("250.1.1.1", 8334);
addrman.Add(CAddress(addr1_port, NODE_NONE), source);
BOOST_CHECK(addrman.size() == 1);
CAddrInfo addr_ret2 = addrman.Select();
BOOST_CHECK(addr_ret2.ToString() == "250.1.1.1:8333");
// Test 8: Add same IP but diff port to tried table, it doesn't get added.
// Perhaps this is not ideal behavior but it is the current behavior.
addrman.Good(CAddress(addr1_port, NODE_NONE));
BOOST_CHECK(addrman.size() == 1);
bool newOnly = true;
CAddrInfo addr_ret3 = addrman.Select(newOnly);
BOOST_CHECK(addr_ret3.ToString() == "250.1.1.1:8333");
}
BOOST_AUTO_TEST_CASE(addrman_select)
{
CAddrManTest addrman;
// Set addrman addr placement to be deterministic.
addrman.MakeDeterministic();
CNetAddr source = ResolveIP("252.2.2.2");
// Test 9: Select from new with 1 addr in new.
CService addr1 = ResolveService("250.1.1.1", 8333);
addrman.Add(CAddress(addr1, NODE_NONE), source);
BOOST_CHECK(addrman.size() == 1);
bool newOnly = true;
CAddrInfo addr_ret1 = addrman.Select(newOnly);
BOOST_CHECK(addr_ret1.ToString() == "250.1.1.1:8333");
// Test 10: move addr to tried, select from new expected nothing returned.
addrman.Good(CAddress(addr1, NODE_NONE));
BOOST_CHECK(addrman.size() == 1);
CAddrInfo addr_ret2 = addrman.Select(newOnly);
BOOST_CHECK(addr_ret2.ToString() == "[::]:0");
CAddrInfo addr_ret3 = addrman.Select();
BOOST_CHECK(addr_ret3.ToString() == "250.1.1.1:8333");
BOOST_CHECK(addrman.size() == 1);
// Add three addresses to new table.
CService addr2 = ResolveService("250.3.1.1", 8333);
CService addr3 = ResolveService("250.3.2.2", 4244);
CService addr4 = ResolveService("250.3.3.3", 4244);
addrman.Add(CAddress(addr2, NODE_NONE), ResolveService("250.3.1.1", 8333));
addrman.Add(CAddress(addr3, NODE_NONE), ResolveService("250.3.1.1", 8333));
addrman.Add(CAddress(addr4, NODE_NONE), ResolveService("250.4.1.1", 8333));
// Add three addresses to tried table.
CService addr5 = ResolveService("250.4.4.4", 8333);
CService addr6 = ResolveService("250.4.5.5", 7777);
CService addr7 = ResolveService("250.4.6.6", 8333);
addrman.Add(CAddress(addr5, NODE_NONE), ResolveService("250.3.1.1", 8333));
addrman.Good(CAddress(addr5, NODE_NONE));
addrman.Add(CAddress(addr6, NODE_NONE), ResolveService("250.3.1.1", 8333));
addrman.Good(CAddress(addr6, NODE_NONE));
addrman.Add(CAddress(addr7, NODE_NONE), ResolveService("250.1.1.3", 8333));
addrman.Good(CAddress(addr7, NODE_NONE));
// Test 11: 6 addrs + 1 addr from last test = 7.
BOOST_CHECK(addrman.size() == 7);
// Test 12: Select pulls from new and tried regardless of port number.
BOOST_CHECK(addrman.Select().ToString() == "250.4.6.6:8333");
BOOST_CHECK(addrman.Select().ToString() == "250.3.2.2:4244");
BOOST_CHECK(addrman.Select().ToString() == "250.3.3.3:4244");
BOOST_CHECK(addrman.Select().ToString() == "250.4.4.4:8333");
}
BOOST_AUTO_TEST_CASE(addrman_new_collisions)
{
CAddrManTest addrman;
// Set addrman addr placement to be deterministic.
addrman.MakeDeterministic();
CNetAddr source = ResolveIP("252.2.2.2");
BOOST_CHECK(addrman.size() == 0);
for (unsigned int i = 1; i < 18; i++) {
CService addr = ResolveService("250.1.1." + boost::to_string(i));
addrman.Add(CAddress(addr, NODE_NONE), source);
//Test 13: No collision in new table yet.
BOOST_CHECK(addrman.size() == i);
}
//Test 14: new table collision!
CService addr1 = ResolveService("250.1.1.18");
addrman.Add(CAddress(addr1, NODE_NONE), source);
BOOST_CHECK(addrman.size() == 17);
CService addr2 = ResolveService("250.1.1.19");
addrman.Add(CAddress(addr2, NODE_NONE), source);
BOOST_CHECK(addrman.size() == 18);
}
BOOST_AUTO_TEST_CASE(addrman_tried_collisions)
{
CAddrManTest addrman;
// Set addrman addr placement to be deterministic.
addrman.MakeDeterministic();
CNetAddr source = ResolveIP("252.2.2.2");
BOOST_CHECK(addrman.size() == 0);
for (unsigned int i = 1; i < 80; i++) {
CService addr = ResolveService("250.1.1." + boost::to_string(i));
addrman.Add(CAddress(addr, NODE_NONE), source);
addrman.Good(CAddress(addr, NODE_NONE));
//Test 15: No collision in tried table yet.
BOOST_TEST_MESSAGE(addrman.size());
BOOST_CHECK(addrman.size() == i);
}
//Test 16: tried table collision!
CService addr1 = ResolveService("250.1.1.80");
addrman.Add(CAddress(addr1, NODE_NONE), source);
BOOST_CHECK(addrman.size() == 79);
CService addr2 = ResolveService("250.1.1.81");
addrman.Add(CAddress(addr2, NODE_NONE), source);
BOOST_CHECK(addrman.size() == 80);
}
BOOST_AUTO_TEST_CASE(addrman_find)
{
CAddrManTest addrman;
// Set addrman addr placement to be deterministic.
addrman.MakeDeterministic();
BOOST_CHECK(addrman.size() == 0);
CAddress addr1 = CAddress(ResolveService("250.1.2.1", 8333), NODE_NONE);
CAddress addr2 = CAddress(ResolveService("250.1.2.1", 4244), NODE_NONE);
CAddress addr3 = CAddress(ResolveService("251.255.2.1", 8333), NODE_NONE);
CNetAddr source1 = ResolveIP("250.1.2.1");
CNetAddr source2 = ResolveIP("250.1.2.2");
addrman.Add(addr1, source1);
addrman.Add(addr2, source2);
addrman.Add(addr3, source1);
// Test 17: ensure Find returns an IP matching what we searched on.
CAddrInfo* info1 = addrman.Find(addr1);
BOOST_CHECK(info1);
if (info1)
BOOST_CHECK(info1->ToString() == "250.1.2.1:8333");
// Test 18; Find does not discriminate by port number.
CAddrInfo* info2 = addrman.Find(addr2);
BOOST_CHECK(info2);
if (info2)
BOOST_CHECK(info2->ToString() == info1->ToString());
// Test 19: Find returns another IP matching what we searched on.
CAddrInfo* info3 = addrman.Find(addr3);
BOOST_CHECK(info3);
if (info3)
BOOST_CHECK(info3->ToString() == "251.255.2.1:8333");
}
BOOST_AUTO_TEST_CASE(addrman_create)
{
CAddrManTest addrman;
// Set addrman addr placement to be deterministic.
addrman.MakeDeterministic();
BOOST_CHECK(addrman.size() == 0);
CAddress addr1 = CAddress(ResolveService("250.1.2.1", 8333), NODE_NONE);
CNetAddr source1 = ResolveIP("250.1.2.1");
int nId;
CAddrInfo* pinfo = addrman.Create(addr1, source1, &nId);
// Test 20: The result should be the same as the input addr.
BOOST_CHECK(pinfo->ToString() == "250.1.2.1:8333");
CAddrInfo* info2 = addrman.Find(addr1);
BOOST_CHECK(info2->ToString() == "250.1.2.1:8333");
}
BOOST_AUTO_TEST_CASE(addrman_delete)
{
CAddrManTest addrman;
// Set addrman addr placement to be deterministic.
addrman.MakeDeterministic();
BOOST_CHECK(addrman.size() == 0);
CAddress addr1 = CAddress(ResolveService("250.1.2.1", 8333), NODE_NONE);
CNetAddr source1 = ResolveIP("250.1.2.1");
int nId;
addrman.Create(addr1, source1, &nId);
// Test 21: Delete should actually delete the addr.
BOOST_CHECK(addrman.size() == 1);
addrman.Delete(nId);
BOOST_CHECK(addrman.size() == 0);
CAddrInfo* info2 = addrman.Find(addr1);
BOOST_CHECK(info2 == NULL);
}
BOOST_AUTO_TEST_CASE(addrman_getaddr)
{
CAddrManTest addrman;
// Set addrman addr placement to be deterministic.
addrman.MakeDeterministic();
// Test 22: Sanity check, GetAddr should never return anything if addrman
// is empty.
BOOST_CHECK(addrman.size() == 0);
vector<CAddress> vAddr1 = addrman.GetAddr();
BOOST_CHECK(vAddr1.size() == 0);
CAddress addr1 = CAddress(ResolveService("250.250.2.1", 8333), NODE_NONE);
addr1.nTime = GetAdjustedTime(); // Set time so isTerrible = false
CAddress addr2 = CAddress(ResolveService("250.251.2.2", 4244), NODE_NONE);
addr2.nTime = GetAdjustedTime();
CAddress addr3 = CAddress(ResolveService("251.252.2.3", 8333), NODE_NONE);
addr3.nTime = GetAdjustedTime();
CAddress addr4 = CAddress(ResolveService("252.253.3.4", 8333), NODE_NONE);
addr4.nTime = GetAdjustedTime();
CAddress addr5 = CAddress(ResolveService("252.254.4.5", 8333), NODE_NONE);
addr5.nTime = GetAdjustedTime();
CNetAddr source1 = ResolveIP("250.1.2.1");
CNetAddr source2 = ResolveIP("250.2.3.3");
// Test 23: Ensure GetAddr works with new addresses.
addrman.Add(addr1, source1);
addrman.Add(addr2, source2);
addrman.Add(addr3, source1);
addrman.Add(addr4, source2);
addrman.Add(addr5, source1);
// GetAddr returns 23% of addresses, 23% of 5 is 1 rounded down.
BOOST_CHECK(addrman.GetAddr().size() == 1);
// Test 24: Ensure GetAddr works with new and tried addresses.
addrman.Good(CAddress(addr1, NODE_NONE));
addrman.Good(CAddress(addr2, NODE_NONE));
BOOST_CHECK(addrman.GetAddr().size() == 1);
// Test 25: Ensure GetAddr still returns 23% when addrman has many addrs.
for (unsigned int i = 1; i < (8 * 256); i++) {
int octet1 = i % 256;
int octet2 = (i / 256) % 256;
int octet3 = (i / (256 * 2)) % 256;
string strAddr = boost::to_string(octet1) + "." + boost::to_string(octet2) + "." + boost::to_string(octet3) + ".23";
CAddress addr = CAddress(ResolveService(strAddr), NODE_NONE);
// Ensure that for all addrs in addrman, isTerrible == false.
addr.nTime = GetAdjustedTime();
addrman.Add(addr, ResolveIP(strAddr));
if (i % 8 == 0)
addrman.Good(addr);
}
vector<CAddress> vAddr = addrman.GetAddr();
size_t percent23 = (addrman.size() * 23) / 100;
BOOST_CHECK(vAddr.size() == percent23);
BOOST_CHECK(vAddr.size() == 461);
// (Addrman.size() < number of addresses added) due to address collisons.
BOOST_CHECK(addrman.size() == 2007);
}
BOOST_AUTO_TEST_CASE(caddrinfo_get_tried_bucket)
{
CAddrManTest addrman;
// Set addrman addr placement to be deterministic.
addrman.MakeDeterministic();
CAddress addr1 = CAddress(ResolveService("250.1.1.1", 8333), NODE_NONE);
CAddress addr2 = CAddress(ResolveService("250.1.1.1", 4244), NODE_NONE);
CNetAddr source1 = ResolveIP("250.1.1.1");
CAddrInfo info1 = CAddrInfo(addr1, source1);
uint256 nKey1 = (uint256)(CHashWriter(SER_GETHASH, 0) << 1).GetHash();
uint256 nKey2 = (uint256)(CHashWriter(SER_GETHASH, 0) << 2).GetHash();
BOOST_CHECK(info1.GetTriedBucket(nKey1) == 40);
// Test 26: Make sure key actually randomizes bucket placement. A fail on
// this test could be a security issue.
BOOST_CHECK(info1.GetTriedBucket(nKey1) != info1.GetTriedBucket(nKey2));
// Test 27: Two addresses with same IP but different ports can map to
// different buckets because they have different keys.
CAddrInfo info2 = CAddrInfo(addr2, source1);
BOOST_CHECK(info1.GetKey() != info2.GetKey());
BOOST_CHECK(info1.GetTriedBucket(nKey1) != info2.GetTriedBucket(nKey1));
set<int> buckets;
for (int i = 0; i < 255; i++) {
CAddrInfo infoi = CAddrInfo(
CAddress(ResolveService("250.1.1." + boost::to_string(i)), NODE_NONE),
ResolveIP("250.1.1." + boost::to_string(i)));
int bucket = infoi.GetTriedBucket(nKey1);
buckets.insert(bucket);
}
// Test 28: IP addresses in the same group (\16 prefix for IPv4) should
// never get more than 8 buckets
BOOST_CHECK(buckets.size() == 8);
buckets.clear();
for (int j = 0; j < 255; j++) {
CAddrInfo infoj = CAddrInfo(
CAddress(ResolveService("250." + boost::to_string(j) + ".1.1"), NODE_NONE),
ResolveIP("250." + boost::to_string(j) + ".1.1"));
int bucket = infoj.GetTriedBucket(nKey1);
buckets.insert(bucket);
}
// Test 29: IP addresses in the different groups should map to more than
// 8 buckets.
BOOST_CHECK(buckets.size() == 160);
}
BOOST_AUTO_TEST_CASE(caddrinfo_get_new_bucket)
{
CAddrManTest addrman;
// Set addrman addr placement to be deterministic.
addrman.MakeDeterministic();
CAddress addr1 = CAddress(ResolveService("250.1.2.1", 8333), NODE_NONE);
CAddress addr2 = CAddress(ResolveService("250.1.2.1", 4244), NODE_NONE);
CNetAddr source1 = ResolveIP("250.1.2.1");
CAddrInfo info1 = CAddrInfo(addr1, source1);
uint256 nKey1 = (uint256)(CHashWriter(SER_GETHASH, 0) << 1).GetHash();
uint256 nKey2 = (uint256)(CHashWriter(SER_GETHASH, 0) << 2).GetHash();
BOOST_CHECK(info1.GetNewBucket(nKey1) == 786);
// Test 30: Make sure key actually randomizes bucket placement. A fail on
// this test could be a security issue.
BOOST_CHECK(info1.GetNewBucket(nKey1) != info1.GetNewBucket(nKey2));
// Test 31: Ports should not effect bucket placement in the addr
CAddrInfo info2 = CAddrInfo(addr2, source1);
BOOST_CHECK(info1.GetKey() != info2.GetKey());
BOOST_CHECK(info1.GetNewBucket(nKey1) == info2.GetNewBucket(nKey1));
set<int> buckets;
for (int i = 0; i < 255; i++) {
CAddrInfo infoi = CAddrInfo(
CAddress(ResolveService("250.1.1." + boost::to_string(i)), NODE_NONE),
ResolveIP("250.1.1." + boost::to_string(i)));
int bucket = infoi.GetNewBucket(nKey1);
buckets.insert(bucket);
}
// Test 32: IP addresses in the same group (\16 prefix for IPv4) should
// always map to the same bucket.
BOOST_CHECK(buckets.size() == 1);
buckets.clear();
for (int j = 0; j < 4 * 255; j++) {
CAddrInfo infoj = CAddrInfo(CAddress(
ResolveService(
boost::to_string(250 + (j / 255)) + "." + boost::to_string(j % 256) + ".1.1"), NODE_NONE),
ResolveIP("251.4.1.1"));
int bucket = infoj.GetNewBucket(nKey1);
buckets.insert(bucket);
}
// Test 33: IP addresses in the same source groups should map to no more
// than 64 buckets.
BOOST_CHECK(buckets.size() <= 64);
buckets.clear();
for (int p = 0; p < 255; p++) {
CAddrInfo infoj = CAddrInfo(
CAddress(ResolveService("250.1.1.1"), NODE_NONE),
ResolveIP("250." + boost::to_string(p) + ".1.1"));
int bucket = infoj.GetNewBucket(nKey1);
buckets.insert(bucket);
}
// Test 34: IP addresses in the different source groups should map to more
// than 64 buckets.
BOOST_CHECK(buckets.size() > 64);
}
BOOST_AUTO_TEST_SUITE_END() | {'content_hash': '92489dbb1aaae7ddbfb2a78aa66c5cb4', 'timestamp': '', 'source': 'github', 'line_count': 534, 'max_line_length': 134, 'avg_line_length': 33.674157303370784, 'alnum_prop': 0.6400289178066956, 'repo_name': 'ArcticCore/arcticcoin', 'id': '79a2f3ef2282d37d95cf91a60eafe870c39c109a', 'size': '18339', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/test/addrman_tests.cpp', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'C', 'bytes': '1290778'}, {'name': 'C++', 'bytes': '5297805'}, {'name': 'CSS', 'bytes': '41859'}, {'name': 'HTML', 'bytes': '50621'}, {'name': 'Java', 'bytes': '2100'}, {'name': 'M4', 'bytes': '147962'}, {'name': 'Makefile', 'bytes': '97720'}, {'name': 'Objective-C', 'bytes': '3222'}, {'name': 'Objective-C++', 'bytes': '7232'}, {'name': 'Python', 'bytes': '706830'}, {'name': 'QMake', 'bytes': '2060'}, {'name': 'Roff', 'bytes': '3874'}, {'name': 'Shell', 'bytes': '35895'}]} |
Based on [UberGallery/ubergallery](https://github.com/UberGallery/ubergallery/), but written in [Go](https://golang.org/) for performance
## Compatibility
Written and tested with Go 1.5. I am not supporting earlier versions, even though they might work.
Running in production on a Debian Stretch, ARM7 server at [Scaleway](https://www.scaleway.com/).
## License
As the original project, this project is licensed MIT.
| {'content_hash': '11498a232c0ae5dbeebe9afc7c8b1790', 'timestamp': '', 'source': 'github', 'line_count': 9, 'max_line_length': 137, 'avg_line_length': 47.0, 'alnum_prop': 0.7635933806146572, 'repo_name': 'EtienneBruines/UberGallery-go', 'id': 'd209e87a11cfbdb3f102d2ed89f237cd5feee2f7', 'size': '440', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'README.md', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Go', 'bytes': '6083'}, {'name': 'HTML', 'bytes': '1350'}]} |
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>statsmodels.discrete.discrete_model.MultinomialResults.remove_data — statsmodels 0.8.0 documentation</title>
<link rel="stylesheet" href="../_static/nature.css" type="text/css" />
<link rel="stylesheet" href="../_static/pygments.css" type="text/css" />
<script type="text/javascript">
var DOCUMENTATION_OPTIONS = {
URL_ROOT: '../',
VERSION: '0.8.0',
COLLAPSE_INDEX: false,
FILE_SUFFIX: '.html',
HAS_SOURCE: true,
SOURCELINK_SUFFIX: '.txt'
};
</script>
<script type="text/javascript" src="../_static/jquery.js"></script>
<script type="text/javascript" src="../_static/underscore.js"></script>
<script type="text/javascript" src="../_static/doctools.js"></script>
<link rel="shortcut icon" href="../_static/statsmodels_hybi_favico.ico"/>
<link rel="author" title="About these documents" href="../about.html" />
<link rel="index" title="Index" href="../genindex.html" />
<link rel="search" title="Search" href="../search.html" />
<link rel="next" title="statsmodels.discrete.discrete_model.MultinomialResults.resid_misclassified" href="statsmodels.discrete.discrete_model.MultinomialResults.resid_misclassified.html" />
<link rel="prev" title="statsmodels.discrete.discrete_model.MultinomialResults.pvalues" href="statsmodels.discrete.discrete_model.MultinomialResults.pvalues.html" />
<link rel="stylesheet" href="../_static/examples.css" type="text/css" />
<link rel="stylesheet" href="../_static/facebox.css" type="text/css" />
<script type="text/javascript" src="../_static/scripts.js">
</script>
<script type="text/javascript" src="../_static/facebox.js">
</script>
</head>
<body role="document">
<div class="headerwrap">
<div class = "header">
<a href = "../index.html">
<img src="../_static/statsmodels_hybi_banner.png" alt="Logo"
style="padding-left: 15px"/></a>
</div>
</div>
<div class="related" role="navigation" aria-label="related navigation">
<h3>Navigation</h3>
<ul>
<li class="right" style="margin-right: 10px">
<a href="../genindex.html" title="General Index"
accesskey="I">index</a></li>
<li class="right" >
<a href="../py-modindex.html" title="Python Module Index"
>modules</a> |</li>
<li class="right" >
<a href="statsmodels.discrete.discrete_model.MultinomialResults.resid_misclassified.html" title="statsmodels.discrete.discrete_model.MultinomialResults.resid_misclassified"
accesskey="N">next</a> |</li>
<li class="right" >
<a href="statsmodels.discrete.discrete_model.MultinomialResults.pvalues.html" title="statsmodels.discrete.discrete_model.MultinomialResults.pvalues"
accesskey="P">previous</a> |</li>
<li><a href ="../install.html">Install</a></li> |
<li><a href="https://groups.google.com/group/pystatsmodels?hl=en">Support</a></li> |
<li><a href="https://github.com/statsmodels/statsmodels/issues">Bugs</a></li> |
<li><a href="../dev/index.html">Develop</a></li> |
<li><a href="../examples/index.html">Examples</a></li> |
<li><a href="../faq.html">FAQ</a></li> |
<li class="nav-item nav-item-1"><a href="../discretemod.html" >Regression with Discrete Dependent Variable</a> |</li>
<li class="nav-item nav-item-2"><a href="statsmodels.discrete.discrete_model.MultinomialResults.html" accesskey="U">statsmodels.discrete.discrete_model.MultinomialResults</a> |</li>
</ul>
</div>
<div class="document">
<div class="documentwrapper">
<div class="bodywrapper">
<div class="body" role="main">
<div class="section" id="statsmodels-discrete-discrete-model-multinomialresults-remove-data">
<h1>statsmodels.discrete.discrete_model.MultinomialResults.remove_data<a class="headerlink" href="#statsmodels-discrete-discrete-model-multinomialresults-remove-data" title="Permalink to this headline">¶</a></h1>
<dl class="method">
<dt id="statsmodels.discrete.discrete_model.MultinomialResults.remove_data">
<code class="descclassname">MultinomialResults.</code><code class="descname">remove_data</code><span class="sig-paren">(</span><span class="sig-paren">)</span><a class="headerlink" href="#statsmodels.discrete.discrete_model.MultinomialResults.remove_data" title="Permalink to this definition">¶</a></dt>
<dd><p>remove data arrays, all nobs arrays from result and model</p>
<p>This reduces the size of the instance, so it can be pickled with less
memory. Currently tested for use with predict from an unpickled
results and model instance.</p>
<div class="admonition warning">
<p class="first admonition-title">Warning</p>
<p class="last">Since data and some intermediate results have been removed
calculating new statistics that require them will raise exceptions.
The exception will occur the first time an attribute is accessed
that has been set to None.</p>
</div>
<p>Not fully tested for time series models, tsa, and might delete too much
for prediction or not all that would be possible.</p>
<p>The lists of arrays to delete are maintained as attributes of
the result and model instance, except for cached values. These
lists could be changed before calling remove_data.</p>
<p>The attributes to remove are named in:</p>
<dl class="docutils">
<dt>model._data_attr <span class="classifier-delimiter">:</span> <span class="classifier">arrays attached to both the model instance</span></dt>
<dd>and the results instance with the same attribute name.</dd>
<dt>result.data_in_cache <span class="classifier-delimiter">:</span> <span class="classifier">arrays that may exist as values in</span></dt>
<dd>result._cache (TODO : should privatize name)</dd>
<dt>result._data_attr_model <span class="classifier-delimiter">:</span> <span class="classifier">arrays attached to the model</span></dt>
<dd>instance but not to the results instance</dd>
</dl>
</dd></dl>
</div>
</div>
</div>
</div>
<div class="sphinxsidebar" role="navigation" aria-label="main navigation">
<div class="sphinxsidebarwrapper">
<h4>Previous topic</h4>
<p class="topless"><a href="statsmodels.discrete.discrete_model.MultinomialResults.pvalues.html"
title="previous chapter">statsmodels.discrete.discrete_model.MultinomialResults.pvalues</a></p>
<h4>Next topic</h4>
<p class="topless"><a href="statsmodels.discrete.discrete_model.MultinomialResults.resid_misclassified.html"
title="next chapter">statsmodels.discrete.discrete_model.MultinomialResults.resid_misclassified</a></p>
<div role="note" aria-label="source link">
<h3>This Page</h3>
<ul class="this-page-menu">
<li><a href="../_sources/generated/statsmodels.discrete.discrete_model.MultinomialResults.remove_data.rst.txt"
rel="nofollow">Show Source</a></li>
</ul>
</div>
<div id="searchbox" style="display: none" role="search">
<h3>Quick search</h3>
<form class="search" action="../search.html" method="get">
<div><input type="text" name="q" /></div>
<div><input type="submit" value="Go" /></div>
<input type="hidden" name="check_keywords" value="yes" />
<input type="hidden" name="area" value="default" />
</form>
</div>
<script type="text/javascript">$('#searchbox').show(0);</script>
</div>
</div>
<div class="clearer"></div>
</div>
<div class="footer" role="contentinfo">
© Copyright 2009-2017, Josef Perktold, Skipper Seabold, Jonathan Taylor, statsmodels-developers.
Created using <a href="http://sphinx-doc.org/">Sphinx</a> 1.5.3.
</div>
</body>
</html> | {'content_hash': 'a59ef62e580db7d94727e5c8dd66bb84', 'timestamp': '', 'source': 'github', 'line_count': 163, 'max_line_length': 303, 'avg_line_length': 49.484662576687114, 'alnum_prop': 0.674435903793702, 'repo_name': 'statsmodels/statsmodels.github.io', 'id': 'c0ed9fc99e863978967e91ccb0e5c0917128446a', 'size': '8069', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': '0.8.0/generated/statsmodels.discrete.discrete_model.MultinomialResults.remove_data.html', 'mode': '33188', 'license': 'bsd-3-clause', 'language': []} |
#!/usr/bin/php -q
<?php
// Copyright 2014 CloudHarmony Inc.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
/**
* saves results based on the arguments defined in ../run.sh
*/
require_once(dirname(__FILE__) . '/GeekbenchTest.php');
require_once(dirname(__FILE__) . '/save/BenchmarkDb.php');
$status = 1;
$args = parse_args(array('iteration:', 'nostore_html', 'nostore_json', 'nostore_rrd', 'nostore_text', 'v' => 'verbose'), NULL, 'save_');
// get result directories => each directory stores 1 iteration of results
$dirs = array();
$dir = count($argv) > 1 && is_dir($argv[count($argv) - 1]) ? $argv[count($argv) - 1] : trim(shell_exec('pwd'));
if (is_dir(sprintf('%s/1', $dir))) {
$i = 1;
while(is_dir($sdir = sprintf('%s/%d', $dir, $i++))) $dirs[] = $sdir;
}
else $dirs[] = $dir;
if ($db =& BenchmarkDb::getDb()) {
// get results from each directory
foreach($dirs as $i => $dir) {
$test = new GeekbenchTest($dir);
$iteration = isset($args['iteration']) && preg_match('/([0-9]+)/', $args['iteration'], $m) ? $m[1]*1 : $i + 1;
if ($results = $test->getResults()) {
$results['iteration'] = $iteration;
print_msg(sprintf('Saving results in directory %s', $dir), isset($args['verbose']), __FILE__, __LINE__);
foreach(array('nostore_html' => 'geekbench.html', 'nostore_json' => 'geekbench.json', 'nostore_rrd' => 'collectd-rrd.zip', 'nostore_text' => 'geekbench.txt') as $arg => $file) {
$file = sprintf('%s/%s', $dir, $file);
if (!isset($args[$arg]) && file_exists($file)) {
$pieces = explode('_', $arg);
$col = $arg == 'nostore_rrd' ? 'collectd_rrd' : sprintf('results_%s', $pieces[count($pieces) - 1]);
$saved = $db->saveArtifact($file, $col);
if ($saved) print_msg(sprintf('Saved %s successfully', basename($file)), isset($args['verbose']), __FILE__, __LINE__);
else if ($saved === NULL) print_msg(sprintf('Unable to save %s', basename($file)), isset($args['verbose']), __FILE__, __LINE__, TRUE);
else print_msg(sprintf('Artifact %s will not be saved because --store was not specified', basename($file)), isset($args['verbose']), __FILE__, __LINE__);
}
else if (file_exists($file)) print_msg(sprintf('Artifact %s will not be saved because --%s was set', basename($file), $arg), isset($args['verbose']), __FILE__, __LINE__);
}
if ($db->addRow('geekbench', $results)) print_msg(sprintf('Successfully saved test results'), isset($args['verbose']), __FILE__, __LINE__);
else print_msg(sprintf('Failed to save test results'), isset($args['verbose']), __FILE__, __LINE__, TRUE);
}
else print_msg(sprintf('Unable to save results in directory %s - are result files present?', $dir), isset($args['verbose']), __FILE__, __LINE__, TRUE);
}
// finalize saving of results
if ($db->save()) {
print_msg(sprintf('Successfully saved test results from directory %s', $dir), isset($args['verbose']), __FILE__, __LINE__);
$status = 0;
}
else {
print_msg(sprintf('Unable to save test results from directory %s', $dir), isset($args['verbose']), __FILE__, __LINE__, TRUE);
$status = 1;
}
}
exit($status);
?>
| {'content_hash': 'aa673331832bb3eb3e76aec07ae81faa', 'timestamp': '', 'source': 'github', 'line_count': 73, 'max_line_length': 183, 'avg_line_length': 50.54794520547945, 'alnum_prop': 0.6124661246612466, 'repo_name': 'cloudharmony/geekbench3', 'id': '426d299e575d1c179af32603671a35b0fa9c39df', 'size': '3690', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'lib/save.php', 'mode': '33261', 'license': 'apache-2.0', 'language': [{'name': 'PHP', 'bytes': '148449'}, {'name': 'Shell', 'bytes': '34774'}]} |
#ifndef YR_THREADING_H
#define YR_THREADING_H
#if defined(_WIN32) || defined(__CYGWIN__)
#include <windows.h>
#else
#include <sys/stat.h>
#include <pthread.h>
#include <semaphore.h>
#endif
#if defined(_WIN32) || defined(__CYGWIN__)
typedef HANDLE SEMAPHORE;
typedef HANDLE MUTEX;
typedef HANDLE THREAD;
typedef LPTHREAD_START_ROUTINE THREAD_START_ROUTINE;
#else
typedef sem_t* SEMAPHORE;
typedef pthread_mutex_t MUTEX;
typedef pthread_t THREAD;
typedef void *(*THREAD_START_ROUTINE) (void *);
#endif
int mutex_init(
MUTEX* mutex);
void mutex_destroy(
MUTEX* mutex);
void mutex_lock(
MUTEX* mutex);
void mutex_unlock(
MUTEX* mutex);
int semaphore_init(
SEMAPHORE* semaphore,
int value);
void semaphore_destroy(
SEMAPHORE* semaphore);
void semaphore_wait(
SEMAPHORE* semaphore);
void semaphore_release(
SEMAPHORE* semaphore);
int create_thread(
THREAD* thread,
THREAD_START_ROUTINE start_routine,
void* param);
void thread_join(
THREAD* thread);
#endif
| {'content_hash': 'e1892f6b05504714d4d4217779e11c93', 'timestamp': '', 'source': 'github', 'line_count': 65, 'max_line_length': 52, 'avg_line_length': 15.753846153846155, 'alnum_prop': 0.7021484375, 'repo_name': 'Security513/yara', 'id': 'f5d32b4ffba70a59d83062a4f71956b38ef2a508', 'size': '1612', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'threading.h', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'C', 'bytes': '2433994'}, {'name': 'C++', 'bytes': '100025'}, {'name': 'Groff', 'bytes': '4343'}, {'name': 'Lex', 'bytes': '32775'}, {'name': 'Shell', 'bytes': '80'}, {'name': 'Yacc', 'bytes': '69584'}]} |
<?php
declare(strict_types=1);
namespace Kreait\Firebase\Tests\Unit\Value;
use Kreait\Firebase\Exception\InvalidArgumentException;
use Kreait\Firebase\Value\ClearTextPassword;
use PHPUnit\Framework\TestCase;
/**
* @internal
*/
final class ClearTextPasswordTest extends TestCase
{
/**
* @dataProvider validValues
*/
public function testWithValidValue(mixed $value): void
{
$password = ClearTextPassword::fromString($value)->value;
$this->assertSame($value, $password);
}
/**
* @dataProvider invalidValues
*/
public function testWithInvalidValue(mixed $value): void
{
$this->expectException(InvalidArgumentException::class);
ClearTextPassword::fromString($value);
}
/**
* @return array<string, array<string>>
*/
public function validValues(): array
{
return [
'long enough' => ['long enough'],
];
}
/**
* @return array<string, array<string>>
*/
public function invalidValues(): array
{
return [
'empty string' => [''],
'less than 6 chars' => ['short'],
];
}
}
| {'content_hash': '203049e51d323235538985b5a43f2bc6', 'timestamp': '', 'source': 'github', 'line_count': 55, 'max_line_length': 65, 'avg_line_length': 21.30909090909091, 'alnum_prop': 0.5998293515358362, 'repo_name': 'kreait/firebase-php', 'id': '88befbd02b31576e3d8b09f88f638ba61e578bf7', 'size': '1172', 'binary': False, 'copies': '1', 'ref': 'refs/heads/7.x', 'path': 'tests/Unit/Value/ClearTextPasswordTest.php', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'PHP', 'bytes': '710292'}]} |
NS_ASSUME_NONNULL_BEGIN
typedef NS_ENUM(NSUInteger, OEXTextWeight) {
OEXTextWeightNormal,
OEXTextWeightLight,
OEXTextWeightSemiBold,
OEXTextWeightBold,
OEXTextWeightBoldItalic
// TODO: Add XLight when necessary
};
typedef NS_ENUM(NSUInteger, OEXLetterSpacing) {
OEXLetterSpacingNormal,
OEXLetterSpacingLoose,
OEXLetterSpacingXLoose,
OEXLetterSpacingXXLoose,
OEXLetterSpacingTight,
OEXLetterSpacingXTight,
OEXLetterSpacingXXTight,
};
typedef NS_ENUM(NSUInteger, OEXTextSize) {
OEXTextSizeBase,
OEXTextSizeXXXXLarge,
OEXTextSizeXXXLarge,
OEXTextSizeXXLarge,
OEXTextSizeXLarge,
OEXTextSizeLarge,
OEXTextSizeXXXSmall,
OEXTextSizeXXSmall,
OEXTextSizeXSmall,
OEXTextSizeSmall,
};
// TODO Add line spacing when necessary
@interface OEXTextStyle : NSObject <NSCopying, NSMutableCopying>
- (id)initWithWeight:(OEXTextWeight)weight size:(OEXTextSize)size color:(nullable UIColor*)color NS_DESIGNATED_INITIALIZER;
- (id)init NS_SWIFT_UNAVAILABLE("Use the designated initializer weight:size:color");
+ (CGFloat)pointSizeForTextSize:(OEXTextSize)size;
+(OEXTextSize)textSizeForPointSize:(int)size;
@property (readonly, assign, nonatomic) NSTextAlignment alignment;
@property (readonly, assign, nonatomic) OEXLetterSpacing letterSpacing;
@property (readonly, strong, nonatomic, nullable) UIColor* color;
@property (readonly, assign, nonatomic) NSLineBreakMode lineBreakMode;
@property (readonly, assign, nonatomic) CGFloat paragraphSpacing;
@property (readonly, assign, nonatomic) CGFloat paragraphSpacingBefore;
@property (readonly, assign, nonatomic) OEXTextSize size;
@property (readonly, assign, nonatomic) OEXTextWeight weight;
@property (readonly, nonatomic) NSDictionary<NSString*, id>* attributes;
/// Duplicates the current style but makes it bold if it is not already
@property (readonly, copy, nonatomic) OEXTextStyle*(^withWeight)(OEXTextWeight weight);
/// Duplicates the current style but with the specified font size
@property (readonly, copy, nonatomic) OEXTextStyle*(^withSize)(OEXTextSize size);
/// Duplicates the current style but with the specified color
@property (readonly, copy, nonatomic) OEXTextStyle*(^withColor)(UIColor* color);
/// Controls Dynamic type support. default true
@property (nonatomic) BOOL dynamicTypeSupported;
- (NSAttributedString*)attributedStringWithText:(nullable NSString*)text;
- (NSAttributedString*)markdownStringWithText:(nullable NSString*)text;
@end
@interface OEXMutableTextStyle : OEXTextStyle
- (instancetype)initWithTextStyle:(OEXTextStyle*)style;
@property (assign, nonatomic) NSTextAlignment alignment;
@property (strong, nonatomic, nullable) UIColor* color;
@property (assign, nonatomic) OEXLetterSpacing letterSpacing;
@property (assign, nonatomic) NSLineBreakMode lineBreakMode;
@property (assign, nonatomic) CGFloat paragraphSpacing;
@property (assign, nonatomic) CGFloat paragraphSpacingBefore;
@property (assign, nonatomic) OEXTextSize size;
@property (assign, nonatomic) OEXTextWeight weight;
@end
NS_ASSUME_NONNULL_END
| {'content_hash': '9189583ba4892c2b04c8b6fbdb43f07e', 'timestamp': '', 'source': 'github', 'line_count': 88, 'max_line_length': 123, 'avg_line_length': 35.06818181818182, 'alnum_prop': 0.7906675307841866, 'repo_name': 'edx/edx-app-ios', 'id': '4d8cf5a06e4a27de349517ea0af6f0bdc13e715f', 'size': '3259', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'Source/OEXTextStyle.h', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'C', 'bytes': '13099'}, {'name': 'CSS', 'bytes': '2524'}, {'name': 'HTML', 'bytes': '3194251'}, {'name': 'JavaScript', 'bytes': '1329'}, {'name': 'Objective-C', 'bytes': '1274825'}, {'name': 'Python', 'bytes': '8765'}, {'name': 'Rich Text Format', 'bytes': '623'}, {'name': 'Ruby', 'bytes': '3047'}, {'name': 'Shell', 'bytes': '1043'}, {'name': 'Swift', 'bytes': '2647828'}]} |
using BenchmarkDotNet.Running;
using System;
namespace NickBuhro.Translit.Benchmark
{
public static class Program
{
public static void Main()
{
var summary = BenchmarkRunner.Run<Benchmark>();
Console.WriteLine(summary);
Console.ReadKey();
}
}
}
| {'content_hash': '0c0f72f04d3e05243a7295a79a38dfbf', 'timestamp': '', 'source': 'github', 'line_count': 15, 'max_line_length': 59, 'avg_line_length': 21.266666666666666, 'alnum_prop': 0.5987460815047022, 'repo_name': 'nick-buhro/Translit', 'id': 'e916e18e3240d8219184ecb8d43fc453ef1cbace', 'size': '321', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'NickBuhro.Translit.Benchmark/Program.cs', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Batchfile', 'bytes': '632'}, {'name': 'C#', 'bytes': '601734'}, {'name': 'Smalltalk', 'bytes': '329040'}]} |
package com.krazy.kcfw.common.persistence.dialect.db;
import com.krazy.kcfw.common.persistence.dialect.Dialect;
/**
* @author poplar.yfyang
* @version 1.0 2010-10-10 下午12:31
* @since JDK 1.5
*/
public class DerbyDialect implements Dialect {
@Override
public boolean supportsLimit() {
return false;
}
@Override
public String getLimitString(String sql, int offset, int limit) {
// return getLimitString(sql,offset,Integer.toString(offset),limit,Integer.toString(limit));
throw new UnsupportedOperationException("paged queries not supported");
}
/**
* 将sql变成分页sql语句,提供将offset及limit使用占位符号(placeholder)替换.
* <pre>
* 如mysql
* dialect.getLimitString("select * from user", 12, ":offset",0,":limit") 将返回
* select * from user limit :offset,:limit
* </pre>
*
* @param sql 实际SQL语句
* @param offset 分页开始纪录条数
* @param offsetPlaceholder 分页开始纪录条数-占位符号
* @param limit 分页每页显示纪录条数
* @param limitPlaceholder 分页纪录条数占位符号
* @return 包含占位符的分页sql
*/
public String getLimitString(String sql, int offset,String offsetPlaceholder, int limit, String limitPlaceholder) {
throw new UnsupportedOperationException( "paged queries not supported" );
}
}
| {'content_hash': '4d0e8c59ccbabf88764c4b48b13ce824', 'timestamp': '', 'source': 'github', 'line_count': 42, 'max_line_length': 116, 'avg_line_length': 30.642857142857142, 'alnum_prop': 0.668997668997669, 'repo_name': 'krazychen/kcfw', 'id': 'c8b436b0dd1282f0c15d39724b84cfcb068f482c', 'size': '1550', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/main/java/com/krazy/kcfw/common/persistence/dialect/db/DerbyDialect.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'ASP', 'bytes': '3753'}, {'name': 'ApacheConf', 'bytes': '768'}, {'name': 'Batchfile', 'bytes': '2125'}, {'name': 'CSS', 'bytes': '943445'}, {'name': 'HTML', 'bytes': '2574065'}, {'name': 'Java', 'bytes': '1640255'}, {'name': 'JavaScript', 'bytes': '8808868'}, {'name': 'PHP', 'bytes': '8060'}]} |
module Chewy
class Type
module Import
extend ActiveSupport::Concern
module ClassMethods
# Perform import operation for specified documents.
# Returns true or false depending on success.
#
# UsersIndex::User.import # imports default data set
# UsersIndex::User.import User.active # imports active users
# UsersIndex::User.import [1, 2, 3] # imports users with specified ids
# UsersIndex::User.import users # imports users collection
# UsersIndex::User.import refresh: false # to disable index refreshing after import
# UsersIndex::User.import suffix: Time.now.to_i # imports data to index with specified suffix if such is exists
# UsersIndex::User.import batch_size: 300 # import batch size
#
# See adapters documentation for more details.
#
def import *args
import_options = args.extract_options!
bulk_options = import_options.reject { |k, v| ![:refresh, :suffix].include?(k) }.reverse_merge!(refresh: true)
index.create!(bulk_options.slice(:suffix)) unless index.exists?
ActiveSupport::Notifications.instrument 'import_objects.chewy', type: self do |payload|
adapter.import(*args, import_options) do |action_objects|
indexed_objects = build_root.parent_id && fetch_indexed_objects(action_objects.values.flatten)
body = bulk_body(action_objects, indexed_objects)
errors = bulk(bulk_options.merge(body: body)) if body.any?
fill_payload_import payload, action_objects
fill_payload_errors payload, errors if errors.present?
!errors.present?
end
end
end
# Perform import operation for specified documents.
# Raises Chewy::ImportFailed exception in case of import errors.
#
# UsersIndex::User.import! # imports default data set
# UsersIndex::User.import! User.active # imports active users
# UsersIndex::User.import! [1, 2, 3] # imports users with specified ids
# UsersIndex::User.import! users # imports users collection
# UsersIndex::User.import! refresh: false # to disable index refreshing after import
# UsersIndex::User.import! suffix: Time.now.to_i # imports data to index with specified suffix if such is exists
# UsersIndex::User.import! batch_size: 300 # import batch size
#
# See adapters documentation for more details.
#
def import! *args
errors = nil
subscriber = ActiveSupport::Notifications.subscribe('import_objects.chewy') do |*args|
errors = args.last[:errors]
end
import *args
raise Chewy::ImportFailed.new(self, errors) if errors.present?
true
ensure
ActiveSupport::Notifications.unsubscribe(subscriber) if subscriber
end
# Wraps elasticsearch-ruby client indices bulk method.
# Adds `:suffix` option to bulk import to index with specified suffix.
def bulk options = {}
suffix = options.delete(:suffix)
result = client.bulk options.merge(index: index.build_index_name(suffix: suffix), type: type_name)
Chewy.wait_for_status
extract_errors result
end
private
def bulk_body(action_objects, indexed_objects = nil)
action_objects.flat_map do |action, objects|
method = "#{action}_bulk_entry"
crutches = Chewy::Type::Crutch::Crutches.new self, objects
objects.flat_map { |object| send(method, object, indexed_objects, crutches) }
end
end
def delete_bulk_entry(object, indexed_objects = nil, crutches = nil)
entry = {}
if root_object.id
entry[:_id] = root_object.compose_id(object)
else
entry[:_id] = object.id if object.respond_to?(:id)
entry[:_id] ||= object[:id] || object['id'] if object.is_a?(Hash)
entry[:_id] ||= object
entry[:_id] = entry[:_id].to_s if defined?(BSON) && entry[:_id].is_a?(BSON::ObjectId)
end
if root_object.parent_id
existing_object = entry[:_id].present? && indexed_objects && indexed_objects[entry[:_id].to_s]
entry.merge!(parent: existing_object[:parent]) if existing_object
end
[{ delete: entry }]
end
def index_bulk_entry(object, indexed_objects = nil, crutches = nil)
entry = {}
if root_object.id
entry[:_id] = root_object.compose_id(object)
else
entry[:_id] = object.id if object.respond_to?(:id)
entry[:_id] ||= object[:id] || object['id'] if object.is_a?(Hash)
entry[:_id] = entry[:_id].to_s if defined?(BSON) && entry[:_id].is_a?(BSON::ObjectId)
end
entry.delete(:_id) if entry[:_id].blank?
if root_object.parent_id
entry[:parent] = root_object.compose_parent(object)
existing_object = entry[:_id].present? && indexed_objects && indexed_objects[entry[:_id].to_s]
end
entry[:data] = object_data(object, crutches)
if existing_object && entry[:parent].to_s != existing_object[:parent]
[{ delete: entry.except(:data).merge(parent: existing_object[:parent]) }, { index: entry }]
else
[{ index: entry }]
end
end
def fill_payload_import payload, action_objects
imported = Hash[action_objects.map { |action, objects| [action, objects.count] }]
imported.each do |action, count|
payload[:import] ||= {}
payload[:import][action] ||= 0
payload[:import][action] += count
end
end
def fill_payload_errors payload, errors
errors.each do |action, errors|
errors.each do |error, documents|
payload[:errors] ||= {}
payload[:errors][action] ||= {}
payload[:errors][action][error] ||= []
payload[:errors][action][error] |= documents
end
end
end
def object_data object, crutches = nil
build_root.compose(object, crutches)[type_name.to_sym]
end
def extract_errors result
result && result['items'].map do |item|
action = item.keys.first.to_sym
data = item.values.first
{action: action, id: data['_id'], error: data['error']} if data['error']
end.compact.group_by { |item| item[:action] }.map do |action, items|
errors = items.group_by { |item| item[:error] }.map do |error, items|
{error => items.map { |item| item[:id] }}
end.reduce(&:merge)
{action => errors}
end.reduce(&:merge) || {}
end
def fetch_indexed_objects(objects)
ids = objects.map { |object| object.respond_to?(:id) ? object.id : object }
result = client.search index: index_name,
type: type_name,
fields: '_parent',
body: { filter: { ids: { values: ids } } },
search_type: 'scan',
scroll: '1m'
indexed_objects = {}
while result = client.scroll(scroll_id: result['_scroll_id'], scroll: '1m') do
break if result['hits']['hits'].empty?
result['hits']['hits'].map do |hit|
parent = hit.has_key?('_parent') ? hit['_parent'] : hit['fields']['_parent']
indexed_objects[hit['_id']] = { parent: parent }
end
end
indexed_objects
end
end
end
end
end
| {'content_hash': '834a395142eed3bea61f5a2d4452c374', 'timestamp': '', 'source': 'github', 'line_count': 194, 'max_line_length': 125, 'avg_line_length': 41.70618556701031, 'alnum_prop': 0.5518477320479546, 'repo_name': 'b2beauty/chewy', 'id': '7a369fed287bb27b9f6caf1f7b831c05a9a179f3', 'size': '8091', 'binary': False, 'copies': '2', 'ref': 'refs/heads/master', 'path': 'lib/chewy/type/import.rb', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Ruby', 'bytes': '421625'}]} |
const params = require('./params');
const format = require('./format');
const parser = require('./parser');
const locale = require('../formatters/_locale.js');
const formatters = {};
/**
* Parse options coming from user-side. Clean it and generate a safe options object for internal use
*
* @param {Object} userDefinedOptions The options object coming from user-side, passed in carbone.render(XML) is used
* @param {<type>} callbackFn The callback function of carbone.render(XML)
* @param {Function} callback The callback of this function
*/
function parseOptions (userDefinedOptions, callbackFn, callback) {
if (typeof(userDefinedOptions) === 'function') {
callbackFn = userDefinedOptions;
userDefinedOptions = {};
}
// define a default complement object with the current date
if (typeof(userDefinedOptions.complement) !== 'object' || userDefinedOptions.complement === null) {
userDefinedOptions.complement = {};
}
if (!userDefinedOptions.complement.now) {
Object.defineProperty(userDefinedOptions.complement, 'now', {
value : (new Date()).toISOString(),
enumerable : false
});
}
// analyze pre-declared variables in the Object "userDefinedOptions"
parser.findVariables(userDefinedOptions.variableStr, function (err, str, variables) {
var _options = {
enum : userDefinedOptions.enum,
currency : {},
lang : (userDefinedOptions.lang || params.lang).toLowerCase(),
timezone : (userDefinedOptions.timezone || params.timezone),
translations : userDefinedOptions.translations || params.translations,
complement : userDefinedOptions.complement,
reportName : userDefinedOptions.reportName,
convertTo : userDefinedOptions.convertTo, // clean by parseConvertTo after
extension : userDefinedOptions.extension,
formatters : formatters,
existingVariables : variables,
hardRefresh : userDefinedOptions.hardRefresh || false,
renderPrefix : userDefinedOptions.renderPrefix
};
var _currency = _options.currency;
var _locale = locale[_options.lang] || locale.en;
var _currencyFromLocale = _locale.currency.code;
_currency.source = userDefinedOptions.currencySource || params.currencySource;
_currency.target = userDefinedOptions.currencyTarget || params.currencyTarget;
_currency.rates = userDefinedOptions.currencyRates || params.currencyRates;
if (!_currency.source) {
_currency.source = _currencyFromLocale;
}
if (!_currency.target) {
_currency.target = _currencyFromLocale;
}
return callback(_options, callbackFn);
});
}
/**
* Parse user-defined convertTo object and set options.convertTo with safe values
*
* options.extension must be set
*
* @param {Object} options coming from parseOptions. It updates options.convertTo and generates this object
* {
* extension : 'pdf',
* format : 'writer_pdf_Export' // coming from lib/format.js
* optionsStr : '44,34,76', // only for CSV
* filters : { // only for PDF, JPG, ...
* ReduceImageResolution : true
* }
* }
* @param {Object} userDefinedConvertTo convertTo, coming from user-side
* @return {String} options Return null if no error. Return a string if there is an error.
*/
function parseConvertTo (options, userDefinedConvertTo) {
if (!options.extension) {
return 'options.extension must be set to detect input file type';
}
var _extensionOutput = userDefinedConvertTo;
var _optionsOutput = {};
if (typeof(userDefinedConvertTo) === 'object' && userDefinedConvertTo !== null) {
_extensionOutput = userDefinedConvertTo.formatName;
_optionsOutput = userDefinedConvertTo.formatOptions;
}
// by default, output = input
if (!_extensionOutput || typeof(_extensionOutput) !== 'string') {
_extensionOutput = options.extension;
}
// clean output file extension
_extensionOutput = _extensionOutput.toLowerCase().trim();
// detect if the conversion is possible and return the output format key for LibreOffice
const _fileFormatOutput = checkDocTypeAndOutputExtensions(options.extension, _extensionOutput);
if (_fileFormatOutput === null && (_extensionOutput !== options.extension || options.hardRefresh === true)) {
return `Format "${options.extension}" can't be converted to "${_extensionOutput}".`;
}
// set default output options
// build internal safe convertTo object
options.convertTo = {
extension : _extensionOutput, // Ex. pdf
format : _fileFormatOutput,// Ex. writer_pdf_Export coming from lib/format.js
optionsStr : '', // Ex. '44,34,76' for CSVs
filters : {} // Ex. { ReduceImageResolution : true, Quality : ... } for PDF, jpg, png, ...
};
switch (_extensionOutput) {
case 'csv':
// set options.convertTo.optionsStr and return null of there is no error
return checkAndSetOptionsForCSV(_optionsOutput, options.convertTo);
case 'pdf':
// set options.convertTo.filters and return null of there is no error
return checkAndSetOptionsForPDF(_optionsOutput, options.convertTo);
default:
break;
}
return null;
}
/**
* Parse options of userDefinedCSVOptions coming from user side and update internal options.convertTo
*
* @param {Object} userDefinedCSVOptions The csv options coming from user-side
* https://wiki.openoffice.org/wiki/Documentation/DevGuide/Spreadsheets/Filter_Options
* {
* fieldSeparator : ',',
* textDelimiter : '"',
* characterSet : '76',
* numberOfFirstLine : 1,
* cellFormat : 1
* }
* @param {Object} convertToObj updates convertTo.optionsStr
* @return {String} null of there is no error, a string if there is an error
*/
function checkAndSetOptionsForCSV (userDefinedCSVOptions, convertToObj) {
// By default, it means:
// Separator : ','
// Delimiter : '"'
// Encoding : UTF-8
var _options = [ '44', '34', '76'];
if (typeof(userDefinedCSVOptions) === 'object' && userDefinedCSVOptions !== null) {
_options[0] = (userDefinedCSVOptions.fieldSeparator || ',').charCodeAt(0);
_options[1] = (userDefinedCSVOptions.textDelimiter || '"').charCodeAt(0);
_options[2] = userDefinedCSVOptions.characterSet || '76';
if (userDefinedCSVOptions.numberOfFirstLine) {
_options[3] = userDefinedCSVOptions.numberOfFirstLine;
}
if (userDefinedCSVOptions.cellFormat) {
_options[4] = userDefinedCSVOptions.cellFormat;
}
}
// set options
convertToObj.optionsStr = _options.join(',');
return null;
}
/**
* Parse options of userDefinedPDFFilters coming from user side and update internal options.convertTo
*
* @param {Object} userDefinedPDFFilters The pdf filters like ReduceImageResolution, ...
* @param {Object} convertToObj updates convertTo.optionsStr
* @return {String} null of there is no error, a string if there is an error
*/
function checkAndSetOptionsForPDF (userDefinedPDFFilters, convertToObj) {
if (userDefinedPDFFilters.ReduceImageResolution === undefined) {
// by default, deactivate compression to speed up conversion
userDefinedPDFFilters.ReduceImageResolution = false;
}
convertToObj.filters = userDefinedPDFFilters;
return null;
}
/**
* Verify files extensions type based on the format.js
*
* @param {String} extensionIn extension type coming from the template file.
* @param {String} extensionOut extension type expected to be converted.
* @return {Boolean} Return true if the extensions format are not matching, otherwise false.
*/
function checkDocTypeAndOutputExtensions (extensionIn, extensionOut) {
for (var docType in format) {
if (format[docType][extensionIn] !== undefined
&& format[docType][extensionOut] !== undefined) {
return format[docType][extensionOut].format;
}
}
return null;
}
module.exports = {
formatters,
parseOptions,
parseConvertTo,
checkAndSetOptionsForCSV,
checkAndSetOptionsForPDF,
checkDocTypeAndOutputExtensions
};
| {'content_hash': '17b76f3bf36ab169f03f1c71063260de', 'timestamp': '', 'source': 'github', 'line_count': 198, 'max_line_length': 131, 'avg_line_length': 45.40909090909091, 'alnum_prop': 0.6247358469580692, 'repo_name': 'Ideolys/carbone', 'id': 'ea24f7b8df35993bfba7ba4df0d47ef3b6a004dc', 'size': '8991', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'lib/input.js', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'JavaScript', 'bytes': '626748'}, {'name': 'Makefile', 'bytes': '77'}, {'name': 'Python', 'bytes': '5244'}]} |
<div class="commune_descr limited">
<p>
Cros est
un village
localisé dans le département de Gard en Languedoc-Roussillon. On dénombrait 245 habitants en 2008.</p>
<p>Le nombre de logements, à Cros, se décomposait en 2011 en cinq appartements et 228 maisons soit
un marché relativement équilibré.</p>
<p>Si vous pensez emmenager à Cros, vous pourrez aisément trouver une maison à acheter. </p>
<p>À coté de Cros sont localisées les communes de
<a href="{{VLROOT}}/immobilier/conqueyrac_30093/">Conqueyrac</a> à 8 km, 104 habitants,
<a href="{{VLROOT}}/immobilier/colognac_30087/">Colognac</a> localisée à 4 km, 197 habitants,
<a href="{{VLROOT}}/immobilier/saint-bonnet-de-salendrinque_30236/">Saint-Bonnet-de-Salendrinque</a> à 5 km, 92 habitants,
<a href="{{VLROOT}}/immobilier/lasalle_30140/">Lasalle</a> à 6 km, 1 052 habitants,
<a href="{{VLROOT}}/immobilier/sumene_30325/">Sumène</a> localisée à 8 km, 1 509 habitants,
<a href="{{VLROOT}}/immobilier/montoulieu_34171/">Montoulieu</a> localisée à 8 km, 145 habitants,
entre autres. De plus, Cros est située à seulement 25 km de <a href="{{VLROOT}}/immobilier/ales_30007/">Alès</a>.</p>
</div>
| {'content_hash': '4a9a2953ba1582d44fe9471484754eb7', 'timestamp': '', 'source': 'github', 'line_count': 17, 'max_line_length': 127, 'avg_line_length': 71.17647058823529, 'alnum_prop': 0.7305785123966942, 'repo_name': 'donaldinou/frontend', 'id': '1d2c5e1dd92ac65e03314955e11eb0fb1dd82af8', 'size': '1237', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/Viteloge/CoreBundle/Resources/descriptions/30099.html', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'ApacheConf', 'bytes': '3073'}, {'name': 'CSS', 'bytes': '111338'}, {'name': 'HTML', 'bytes': '58634405'}, {'name': 'JavaScript', 'bytes': '88564'}, {'name': 'PHP', 'bytes': '841919'}]} |
<android.support.v4.view.ViewPager xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/pager"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.sampa.tasksreminder.MainActivity" />
| {'content_hash': '1d9912dcc0a2f864359e7c2f66d6d576', 'timestamp': '', 'source': 'github', 'line_count': 7, 'max_line_length': 93, 'avg_line_length': 45.0, 'alnum_prop': 0.7333333333333333, 'repo_name': 'spatenotte/TasksReminder', 'id': 'daa56800941c5e115f7ba1738e4ce530710168fc', 'size': '315', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'TasksReminder/res/layout/activity_main.xml', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Java', 'bytes': '138198'}]} |
require 'jrb-hdfs_jars.rb'
require "jrb/hdfs/client"
| {'content_hash': '2075dce3458d3070390b62edf072f8ab', 'timestamp': '', 'source': 'github', 'line_count': 2, 'max_line_length': 26, 'avg_line_length': 26.5, 'alnum_prop': 0.7547169811320755, 'repo_name': 'myl2821/jrb-hdfs', 'id': '770a3c5f0f632a896dea964c22ab44e6d5b09369', 'size': '53', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'lib/jrb/hdfs.rb', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Ruby', 'bytes': '5806'}, {'name': 'Shell', 'bytes': '131'}]} |
try:
print 'try....'
r = 10 / 0
print 'result:', r
except ZeroDivisionError, e:
print 'except:', e
finally:
print 'finally...'
print 'END'
try:
print 'try...'
r = 10 / int('a')
print 'result: ', r
except ValueError, e:
print 'ValueError:', e
except ZeroDivisionError, e:
print 'ZeroDivisionError:', e
else:
print 'no error!'
finally:
print 'finally...'
print 'END'
import logging
def foo(s):
return 10 / int(s)
def bar(s):
return foo(s) * 2
def main():
try:
bar('0')
except StandardError, e:
logging.exception(e)
main()
print 'END'
| {'content_hash': '64bfab07bdb5f0d8566dac8646083421', 'timestamp': '', 'source': 'github', 'line_count': 38, 'max_line_length': 33, 'avg_line_length': 16.210526315789473, 'alnum_prop': 0.577922077922078, 'repo_name': 'snownothing/Python', 'id': '69c2f0f2312c12cd88cebf219f380a53818c05bb', 'size': '656', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'liaoxuefeng.com/034-Exception.py', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '25'}, {'name': 'HTML', 'bytes': '1686'}, {'name': 'JavaScript', 'bytes': '803'}, {'name': 'Python', 'bytes': '46982'}]} |
<?php
use Acme\Validation\Capsule\Manager as Validation;
class Validator{
public static function __callStatic($name, $args)
{
// Define the locale and the path to the language directory.
$validation = new Validation('en', APPDIR .'/lang');
// Adding a database connection is optional. Only used for
// the Exists and Unique rules.
$db_config = require APPDIR . 'config/database.php';
$validation->setConnection($db_config);
$validator = $validation->getValidator();
return call_user_func_array(array($validator, $name), $args);
}
} | {'content_hash': 'fd79890e0af6d79607b59567c637c718', 'timestamp': '', 'source': 'github', 'line_count': 18, 'max_line_length': 69, 'avg_line_length': 33.888888888888886, 'alnum_prop': 0.6459016393442623, 'repo_name': 'davinder17s/turbo', 'id': 'f433a5eea1196b5286541af545e91e9d62b45b1f', 'size': '610', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'system/components/eloquent/validator.php', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'ApacheConf', 'bytes': '211'}, {'name': 'PHP', 'bytes': '827705'}]} |
package com.infinityraider.settlercraft.settlement.settler.ai;
import com.infinityraider.settlercraft.settlement.settler.EntitySettler;
import net.minecraft.entity.ai.EntityAIBase;
import net.minecraft.entity.player.EntityPlayer;
public class EntityAITalkToPlayer extends EntityAIBase {
private final EntitySettler settler;
public EntityAITalkToPlayer(EntitySettler settler) {
this.settler = settler;
this.setMutexBits(5);
}
public EntitySettler getSettler() {
return settler;
}
/**
* Returns whether the EntityAIBase should begin execution.
*/
@Override
public boolean shouldExecute() {
if (!this.getSettler().isEntityAlive()) {
return false;
}
else if (this.getSettler().isInWater()) {
return false;
}
else if (!this.getSettler().onGround) {
return false;
}
else if (this.getSettler().velocityChanged) {
return false;
}
else {
EntityPlayer player = this.getSettler().getConversationPartner();
return player != null && (this.getSettler().getDistanceSqToEntity(player) <= 16.0D && player.openContainer != null);
}
}
/**
* Execute a one shot task or start executing a continuous task
*/
@Override
public void startExecuting() {
this.getSettler().getNavigator().clearPathEntity();
}
/**
* Resets the task
*/
@Override
public void resetTask() {
EntityPlayer player = this.getSettler().getConversationPartner();
//Can be null if the container was closed from within the conversation
if(player != null) {
player.closeScreen();
}
}
}
| {'content_hash': 'c83f98e8f912ab8cfa4996d60b352b95', 'timestamp': '', 'source': 'github', 'line_count': 61, 'max_line_length': 128, 'avg_line_length': 28.885245901639344, 'alnum_prop': 0.623155505107832, 'repo_name': 'InfinityRaider/SettlerCraft', 'id': 'd5cab332ea33feb7ad5a3a7d9558416d059668c8', 'size': '1762', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/main/java/com/infinityraider/settlercraft/settlement/settler/ai/EntityAITalkToPlayer.java', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Java', 'bytes': '774285'}]} |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>metacoq-checker: Not compatible 👼</title>
<link rel="shortcut icon" type="image/png" href="../../../../../favicon.png" />
<link href="../../../../../bootstrap.min.css" rel="stylesheet">
<link href="../../../../../bootstrap-custom.css" rel="stylesheet">
<link href="//maxcdn.bootstrapcdn.com/font-awesome/4.2.0/css/font-awesome.min.css" rel="stylesheet">
<script src="../../../../../moment.min.js"></script>
<!-- HTML5 Shim and Respond.js IE8 support of HTML5 elements and media queries -->
<!-- WARNING: Respond.js doesn't work if you view the page via file:// -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/html5shiv/3.7.2/html5shiv.min.js"></script>
<script src="https://oss.maxcdn.com/respond/1.4.2/respond.min.js"></script>
<![endif]-->
</head>
<body>
<div class="container">
<div class="navbar navbar-default" role="navigation">
<div class="container-fluid">
<div class="navbar-header">
<a class="navbar-brand" href="../../../../.."><i class="fa fa-lg fa-flag-checkered"></i> Coq bench</a>
</div>
<div id="navbar" class="collapse navbar-collapse">
<ul class="nav navbar-nav">
<li><a href="../..">clean / released</a></li>
<li class="active"><a href="">8.5.0 / metacoq-checker - 1.0~beta1+8.12</a></li>
</ul>
</div>
</div>
</div>
<div class="article">
<div class="row">
<div class="col-md-12">
<a href="../..">« Up</a>
<h1>
metacoq-checker
<small>
1.0~beta1+8.12
<span class="label label-info">Not compatible 👼</span>
</small>
</h1>
<p>📅 <em><script>document.write(moment("2022-11-24 02:35:00 +0000", "YYYY-MM-DD HH:mm:ss Z").fromNow());</script> (2022-11-24 02:35:00 UTC)</em><p>
<h2>Context</h2>
<pre># Packages matching: installed
# Name # Installed # Synopsis
base-bigarray base
base-num base Num library distributed with the OCaml compiler
base-threads base
base-unix base
camlp5 7.14 Preprocessor-pretty-printer of OCaml
conf-findutils 1 Virtual package relying on findutils
conf-perl 2 Virtual package relying on perl
coq 8.5.0 Formal proof management system
num 0 The Num library for arbitrary-precision integer and rational arithmetic
ocaml 4.03.0 The OCaml compiler (virtual package)
ocaml-base-compiler 4.03.0 Official 4.03.0 release
ocaml-config 1 OCaml Switch Configuration
# opam file:
opam-version: "2.0"
maintainer: "[email protected]"
homepage: "https://metacoq.github.io/metacoq"
dev-repo: "git+https://github.com/MetaCoq/metacoq.git#coq-8.12"
bug-reports: "https://github.com/MetaCoq/metacoq/issues"
authors: ["Abhishek Anand <[email protected]>"
"Simon Boulier <[email protected]>"
"Cyril Cohen <[email protected]>"
"Yannick Forster <[email protected]>"
"Fabian Kunze <[email protected]>"
"Gregory Malecha <[email protected]>"
"Matthieu Sozeau <[email protected]>"
"Nicolas Tabareau <[email protected]>"
"Théo Winterhalter <[email protected]>"
]
license: "MIT"
build: [
["sh" "./configure.sh"]
[make "-j%{jobs}%" "checker"]
]
install: [
[make "-C" "checker" "install"]
]
depends: [
"ocaml" {>= "4.07.1"}
"coq" {>= "8.12" & < "8.13~"}
"coq-equations" { = "1.2.3+8.12" }
"coq-metacoq-template" {= version}
]
synopsis: "Specification of Coq's type theory and reference checker implementation"
description: """
MetaCoq is a meta-programming framework for Coq.
The Checker module provides a complete specification of Coq's typing and conversion
relation along with a reference type-checker that is extracted to a pluging.
This provides a command: `MetaCoq Check [global_reference]` that can be used
to typecheck a Coq definition using the verified type-checker.
"""
url {
src: "https://github.com/MetaCoq/metacoq/archive/v1.0-beta1-8.12.tar.gz"
checksum: "sha256=19fc4475ae81677018e21a1e20503716a47713ec8b2081e7506f5c9390284c7a"
}
</pre>
<h2>Lint</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Dry install 🏜️</h2>
<p>Dry install with the current Coq version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam install -y --show-action coq-metacoq-checker.1.0~beta1+8.12 coq.8.5.0</code></dd>
<dt>Return code</dt>
<dd>5120</dd>
<dt>Output</dt>
<dd><pre>[NOTE] Package coq is already installed (current version is 8.5.0).
The following dependencies couldn't be met:
- coq-metacoq-checker -> ocaml >= 4.07.1
base of this switch (use `--unlock-base' to force)
No solution found, exiting
</pre></dd>
</dl>
<p>Dry install without Coq/switch base, to test if the problem was incompatibility with the current Coq/OCaml version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam remove -y coq; opam install -y --show-action --unlock-base coq-metacoq-checker.1.0~beta1+8.12</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Install dependencies</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>0 s</dd>
</dl>
<h2>Install 🚀</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>0 s</dd>
</dl>
<h2>Installation size</h2>
<p>No files were installed.</p>
<h2>Uninstall 🧹</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Missing removes</dt>
<dd>
none
</dd>
<dt>Wrong removes</dt>
<dd>
none
</dd>
</dl>
</div>
</div>
</div>
<hr/>
<div class="footer">
<p class="text-center">
Sources are on <a href="https://github.com/coq-bench">GitHub</a> © Guillaume Claret 🐣
</p>
</div>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
<script src="../../../../../bootstrap.min.js"></script>
</body>
</html>
| {'content_hash': '5a3446dfb035fee7bd1544a733de5685', 'timestamp': '', 'source': 'github', 'line_count': 181, 'max_line_length': 159, 'avg_line_length': 42.88397790055249, 'alnum_prop': 0.5574594176758567, 'repo_name': 'coq-bench/coq-bench.github.io', 'id': '7734d5b457b3d612f4ab3b6b4c5818dbd58d6675', 'size': '7788', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'clean/Linux-x86_64-4.03.0-2.0.5/released/8.5.0/metacoq-checker/1.0~beta1+8.12.html', 'mode': '33188', 'license': 'mit', 'language': []} |
package commands
import (
"github.com/bogem/nehm/api"
"github.com/bogem/nehm/config"
"github.com/bogem/nehm/downloader"
"github.com/bogem/nehm/logs"
"github.com/bogem/nehm/menu"
"github.com/spf13/cobra"
)
var (
// listCommand is root command for nehm.
listCommand = &cobra.Command{
Use: "nehm",
Short: "List likes from your account, download them, set ID3 tags and add them to iTunes",
Long: "nehm is a console tool, which downloads, sets ID3 tags (and adds to your iTunes library) your SoundCloud likes in convenient way.",
Run: showListOfTracks,
PersistentPreRun: activateVerboseOutput,
}
)
// activateVerboseOutput activates verbose output, if verbose flag is provided.
func activateVerboseOutput(cmd *cobra.Command, args []string) {
if verbose {
logs.EnableInfo()
}
}
func init() {
listCommand.PersistentFlags().BoolVarP(&verbose, "verbose", "v", false, "verbose output")
addDlFolderFlag(listCommand)
addItunesPlaylistFlag(listCommand)
addLimitFlag(listCommand)
addPermalinkFlag(listCommand)
}
func showListOfTracks(cmd *cobra.Command, args []string) {
logs.FEEDBACK.Println("Loading...")
initializeConfig(cmd)
uid := api.UID(config.Get("permalink"))
tm := menu.NewTracksMenu(api.FormFavoritesURL(limit, uid))
downloadTracks := tm.Show()
downloader.NewConfiguredDownloader().DownloadAll(downloadTracks)
}
| {'content_hash': '57751108b36458d6ab6febf94828fceb', 'timestamp': '', 'source': 'github', 'line_count': 49, 'max_line_length': 152, 'avg_line_length': 28.591836734693878, 'alnum_prop': 0.7251962883654532, 'repo_name': 'bogem/nehm', 'id': '75617d0c5a6e735bc4d1280d27e57555dce2058e', 'size': '1566', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'commands/list.go', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Go', 'bytes': '36590'}]} |
Solutions to various Project Euler (projecteuler.net) problems, written in C++, are provided here-in with problem number and problem
description.
| {'content_hash': '656c5be56c8416aaad7714d2df4714cb', 'timestamp': '', 'source': 'github', 'line_count': 2, 'max_line_length': 132, 'avg_line_length': 73.0, 'alnum_prop': 0.8082191780821918, 'repo_name': 'BRutan/Cpp', 'id': 'a9216521a33a4d7fe2932e2c86cd5d0e7f2185d4', 'size': '474', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'Project Euler/README.md', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'C++', 'bytes': '495543'}]} |
<Type Name="NSColorWell" FullName="MonoMac.AppKit.NSColorWell">
<TypeSignature Language="C#" Value="public class NSColorWell : MonoMac.AppKit.NSControl" />
<TypeSignature Language="ILAsm" Value=".class public auto ansi beforefieldinit NSColorWell extends MonoMac.AppKit.NSControl" />
<AssemblyInfo>
<AssemblyName>MonoMac</AssemblyName>
<AssemblyVersion>0.0.0.0</AssemblyVersion>
</AssemblyInfo>
<Base>
<BaseTypeName>MonoMac.AppKit.NSControl</BaseTypeName>
</Base>
<Interfaces />
<Attributes>
<Attribute>
<AttributeName>MonoMac.Foundation.Register("NSColorWell")</AttributeName>
</Attribute>
</Attributes>
<Docs>
<summary>To be added.</summary>
<remarks>To be added.</remarks>
</Docs>
<Members>
<Member MemberName=".ctor">
<MemberSignature Language="C#" Value="public NSColorWell ();" />
<MemberSignature Language="ILAsm" Value=".method public hidebysig specialname rtspecialname instance void .ctor() cil managed" />
<MemberType>Constructor</MemberType>
<AssemblyInfo>
<AssemblyVersion>0.0.0.0</AssemblyVersion>
</AssemblyInfo>
<Attributes>
<Attribute>
<AttributeName>MonoMac.Foundation.Export("init")</AttributeName>
</Attribute>
</Attributes>
<Parameters />
<Docs>
<summary>To be added.</summary>
<remarks>To be added.</remarks>
</Docs>
</Member>
<Member MemberName=".ctor">
<MemberSignature Language="C#" Value="public NSColorWell (MonoMac.Foundation.NSCoder coder);" />
<MemberSignature Language="ILAsm" Value=".method public hidebysig specialname rtspecialname instance void .ctor(class MonoMac.Foundation.NSCoder coder) cil managed" />
<MemberType>Constructor</MemberType>
<AssemblyInfo>
<AssemblyVersion>0.0.0.0</AssemblyVersion>
</AssemblyInfo>
<Attributes>
<Attribute>
<AttributeName>MonoMac.Foundation.Export("initWithCoder:")</AttributeName>
</Attribute>
</Attributes>
<Parameters>
<Parameter Name="coder" Type="MonoMac.Foundation.NSCoder" />
</Parameters>
<Docs>
<param name="coder">The unarchiver object.</param>
<summary>A constructor that initializes the object from the data stored in the unarchiver object.</summary>
<remarks>This constructor is provided to allow the class to be initialized from an unarchiver (for example, during NIB deserialization).</remarks>
</Docs>
</Member>
<Member MemberName=".ctor">
<MemberSignature Language="C#" Value="public NSColorWell (MonoMac.Foundation.NSObjectFlag t);" />
<MemberSignature Language="ILAsm" Value=".method public hidebysig specialname rtspecialname instance void .ctor(class MonoMac.Foundation.NSObjectFlag t) cil managed" />
<MemberType>Constructor</MemberType>
<AssemblyInfo>
<AssemblyVersion>0.0.0.0</AssemblyVersion>
</AssemblyInfo>
<Parameters>
<Parameter Name="t" Type="MonoMac.Foundation.NSObjectFlag" />
</Parameters>
<Docs>
<param name="t">Unused sentinel value, pass NSObjectFlag.Empty.</param>
<summary>Constructor to call on derived classes when the derived class has an [Export] constructor.</summary>
<remarks>
<para>This constructor should be called by derived classes when they are initialized using an [Export] attribute. The argument value is ignore, typically the chaining would look like this:</para>
<example>
<code lang="C#">
public class MyClass : BaseClass {
[Export ("initWithFoo:")]
public MyClass (string foo) : base (NSObjectFlag.Empty)
{
...
}
</code>
</example>
</remarks>
</Docs>
</Member>
<Member MemberName=".ctor">
<MemberSignature Language="C#" Value="public NSColorWell (System.Drawing.RectangleF frameRect);" />
<MemberSignature Language="ILAsm" Value=".method public hidebysig specialname rtspecialname instance void .ctor(valuetype System.Drawing.RectangleF frameRect) cil managed" />
<MemberType>Constructor</MemberType>
<AssemblyInfo>
<AssemblyVersion>0.0.0.0</AssemblyVersion>
</AssemblyInfo>
<Attributes>
<Attribute>
<AttributeName>MonoMac.Foundation.Export("initWithFrame:")</AttributeName>
</Attribute>
</Attributes>
<Parameters>
<Parameter Name="frameRect" Type="System.Drawing.RectangleF" />
</Parameters>
<Docs>
<param name="frameRect">To be added.</param>
<summary>To be added.</summary>
<remarks>To be added.</remarks>
</Docs>
</Member>
<Member MemberName=".ctor">
<MemberSignature Language="C#" Value="public NSColorWell (IntPtr handle);" />
<MemberSignature Language="ILAsm" Value=".method public hidebysig specialname rtspecialname instance void .ctor(native int handle) cil managed" />
<MemberType>Constructor</MemberType>
<AssemblyInfo>
<AssemblyVersion>0.0.0.0</AssemblyVersion>
</AssemblyInfo>
<Parameters>
<Parameter Name="handle" Type="System.IntPtr" />
</Parameters>
<Docs>
<param name="handle">Pointer (handle) to the unmanaged object.</param>
<summary>A constructor used when creating managed representations of unmanaged objects; Called by the runtime.</summary>
<remarks>
<para>This constructor is invoked by the runtime infrastructure (<see cref="M:MonoMac.ObjCRuntime.GetNSObject (System.IntPtr)" />) to create a new managed representation for a pointer to an unmanaged Objective-C object. You should not invoke this method directly, instead you should call the GetNSObject method as it will prevent two instances of a managed object to point to the same native object.</para>
</remarks>
</Docs>
</Member>
<Member MemberName="Activate">
<MemberSignature Language="C#" Value="public virtual void Activate (bool exclusive);" />
<MemberSignature Language="ILAsm" Value=".method public hidebysig newslot virtual instance void Activate(bool exclusive) cil managed" />
<MemberType>Method</MemberType>
<AssemblyInfo>
<AssemblyVersion>0.0.0.0</AssemblyVersion>
</AssemblyInfo>
<Attributes>
<Attribute>
<AttributeName>MonoMac.Foundation.Export("activate:")</AttributeName>
</Attribute>
</Attributes>
<ReturnValue>
<ReturnType>System.Void</ReturnType>
</ReturnValue>
<Parameters>
<Parameter Name="exclusive" Type="System.Boolean" />
</Parameters>
<Docs>
<param name="exclusive">To be added.</param>
<summary>To be added.</summary>
<remarks>To be added.</remarks>
</Docs>
</Member>
<Member MemberName="Bordered">
<MemberSignature Language="C#" Value="public virtual bool Bordered { get; set; }" />
<MemberSignature Language="ILAsm" Value=".property instance bool Bordered" />
<MemberType>Property</MemberType>
<AssemblyInfo>
<AssemblyVersion>0.0.0.0</AssemblyVersion>
</AssemblyInfo>
<Attributes>
<Attribute>
<AttributeName>get: MonoMac.Foundation.Export("isBordered")</AttributeName>
</Attribute>
<Attribute>
<AttributeName>set: MonoMac.Foundation.Export("setBordered:")</AttributeName>
</Attribute>
</Attributes>
<ReturnValue>
<ReturnType>System.Boolean</ReturnType>
</ReturnValue>
<Docs>
<summary>To be added.</summary>
<value>To be added.</value>
<remarks>To be added.</remarks>
</Docs>
</Member>
<Member MemberName="ClassHandle">
<MemberSignature Language="C#" Value="public override IntPtr ClassHandle { get; }" />
<MemberSignature Language="ILAsm" Value=".property instance native int ClassHandle" />
<MemberType>Property</MemberType>
<AssemblyInfo>
<AssemblyVersion>0.0.0.0</AssemblyVersion>
</AssemblyInfo>
<ReturnValue>
<ReturnType>System.IntPtr</ReturnType>
</ReturnValue>
<Docs>
<summary>The handle for this class.</summary>
<value>The pointer to the Objective-C class.</value>
<remarks>Each MonoMac class mirrors an unmanaged Objective-C class. This value contains the pointer to the Objective-C class, it is similar to calling objc_getClass with the object name.</remarks>
</Docs>
</Member>
<Member MemberName="Color">
<MemberSignature Language="C#" Value="public virtual MonoMac.AppKit.NSColor Color { get; set; }" />
<MemberSignature Language="ILAsm" Value=".property instance class MonoMac.AppKit.NSColor Color" />
<MemberType>Property</MemberType>
<AssemblyInfo>
<AssemblyVersion>0.0.0.0</AssemblyVersion>
</AssemblyInfo>
<Attributes>
<Attribute>
<AttributeName>get: MonoMac.Foundation.Export("color")</AttributeName>
</Attribute>
<Attribute>
<AttributeName>set: MonoMac.Foundation.Export("setColor:")</AttributeName>
</Attribute>
</Attributes>
<ReturnValue>
<ReturnType>MonoMac.AppKit.NSColor</ReturnType>
</ReturnValue>
<Docs>
<summary>To be added.</summary>
<value>To be added.</value>
<remarks>To be added.</remarks>
</Docs>
</Member>
<Member MemberName="Deactivate">
<MemberSignature Language="C#" Value="public virtual void Deactivate ();" />
<MemberSignature Language="ILAsm" Value=".method public hidebysig newslot virtual instance void Deactivate() cil managed" />
<MemberType>Method</MemberType>
<AssemblyInfo>
<AssemblyVersion>0.0.0.0</AssemblyVersion>
</AssemblyInfo>
<Attributes>
<Attribute>
<AttributeName>MonoMac.Foundation.Export("deactivate")</AttributeName>
</Attribute>
</Attributes>
<ReturnValue>
<ReturnType>System.Void</ReturnType>
</ReturnValue>
<Parameters />
<Docs>
<summary>To be added.</summary>
<remarks>To be added.</remarks>
</Docs>
</Member>
<Member MemberName="DrawWellInside">
<MemberSignature Language="C#" Value="public virtual void DrawWellInside (System.Drawing.RectangleF insideRect);" />
<MemberSignature Language="ILAsm" Value=".method public hidebysig newslot virtual instance void DrawWellInside(valuetype System.Drawing.RectangleF insideRect) cil managed" />
<MemberType>Method</MemberType>
<AssemblyInfo>
<AssemblyVersion>0.0.0.0</AssemblyVersion>
</AssemblyInfo>
<Attributes>
<Attribute>
<AttributeName>MonoMac.Foundation.Export("drawWellInside:")</AttributeName>
</Attribute>
</Attributes>
<ReturnValue>
<ReturnType>System.Void</ReturnType>
</ReturnValue>
<Parameters>
<Parameter Name="insideRect" Type="System.Drawing.RectangleF" />
</Parameters>
<Docs>
<param name="insideRect">To be added.</param>
<summary>To be added.</summary>
<remarks>To be added.</remarks>
</Docs>
</Member>
<Member MemberName="IsActive">
<MemberSignature Language="C#" Value="public virtual bool IsActive { get; }" />
<MemberSignature Language="ILAsm" Value=".property instance bool IsActive" />
<MemberType>Property</MemberType>
<AssemblyInfo>
<AssemblyVersion>0.0.0.0</AssemblyVersion>
</AssemblyInfo>
<Attributes>
<Attribute>
<AttributeName>get: MonoMac.Foundation.Export("isActive")</AttributeName>
</Attribute>
</Attributes>
<ReturnValue>
<ReturnType>System.Boolean</ReturnType>
</ReturnValue>
<Docs>
<summary>To be added.</summary>
<value>To be added.</value>
<remarks>To be added.</remarks>
</Docs>
</Member>
<Member MemberName="TakeColorFrom">
<MemberSignature Language="C#" Value="public virtual void TakeColorFrom (MonoMac.Foundation.NSObject sender);" />
<MemberSignature Language="ILAsm" Value=".method public hidebysig newslot virtual instance void TakeColorFrom(class MonoMac.Foundation.NSObject sender) cil managed" />
<MemberType>Method</MemberType>
<AssemblyInfo>
<AssemblyVersion>0.0.0.0</AssemblyVersion>
</AssemblyInfo>
<Attributes>
<Attribute>
<AttributeName>MonoMac.Foundation.Export("takeColorFrom:")</AttributeName>
</Attribute>
</Attributes>
<ReturnValue>
<ReturnType>System.Void</ReturnType>
</ReturnValue>
<Parameters>
<Parameter Name="sender" Type="MonoMac.Foundation.NSObject" />
</Parameters>
<Docs>
<param name="sender">To be added.</param>
<summary>To be added.</summary>
<remarks>To be added.</remarks>
</Docs>
</Member>
</Members>
</Type>
| {'content_hash': 'c23e8bb3afbd338fa279b11e48dcd0bc', 'timestamp': '', 'source': 'github', 'line_count': 307, 'max_line_length': 419, 'avg_line_length': 42.306188925081436, 'alnum_prop': 0.6552202032645519, 'repo_name': 'kangaroo/monomac', 'id': 'eaa0648408537d80921d9dbdabc2756294ae5ecb', 'size': '12988', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'docs/en/MonoMac.AppKit/NSColorWell.xml', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'C#', 'bytes': '8582454'}]} |
<?php
namespace PhpDocBlockChecker\Check;
use PhpDocBlockChecker\Config\Config;
use PhpDocBlockChecker\FileInfo;
use PhpDocBlockChecker\Status\FileStatus;
use PhpDocBlockChecker\Status\StatusType\Passed\Passed;
class Checker
{
/**
* @var Config
*/
private $config;
private $checks = [
ClassCheck::class,
MethodCheck::class,
ParamCheck::class,
ReturnCheck::class
];
public function __construct(Config $config)
{
$this->config = $config;
}
/**
* @param FileInfo $fileInfo
* @return FileStatus
*/
public function check(FileInfo $fileInfo)
{
$fileStatus = new FileStatus();
foreach ($this->checks as $check) {
/** @var CheckInterface $check */
$check = new $check($this->config, $fileStatus);
if ($check->enabled()) {
$check->check($fileInfo);
}
}
if (!$fileStatus->hasErrors()) {
$fileStatus->add(new Passed($fileInfo->getFileName()));
}
return $fileStatus;
}
}
| {'content_hash': 'ff549b3429d2c7402ab71fa87c4fe687', 'timestamp': '', 'source': 'github', 'line_count': 50, 'max_line_length': 67, 'avg_line_length': 22.0, 'alnum_prop': 0.57, 'repo_name': 'Block8/php-docblock-checker', 'id': '8482e3dd57a90f88c0d015dcf4f8f7860e3b2996', 'size': '1100', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/Check/Checker.php', 'mode': '33188', 'license': 'bsd-2-clause', 'language': [{'name': 'PHP', 'bytes': '30908'}]} |
package org.openbel.rest.common;
import static java.lang.String.format;
import static org.openbel.rest.common.Objects.*;
import static org.openbel.rest.Util.*;
import static org.openbel.rest.main.*;
import static org.openbel.framework.common.bel.parser.BELParser.*;
import org.openbel.rest.common.Objects;
import org.jongo.*;
import org.openbel.rest.Path;
import org.restlet.resource.Post;
import org.restlet.resource.ServerResource;
import org.openbel.framework.common.model.*;
import org.openbel.framework.common.bel.parser.BELParseResults;
import org.openbel.bel.model.*;
import org.restlet.representation.Representation;
import org.restlet.ext.jackson.JacksonRepresentation;
import org.restlet.data.Status;
import java.util.*;
@Path("/api/v1/lang/validater/term")
public class TermValidater extends ServerResource {
@Post("txt")
public Representation _post(Representation body) {
String txt = textify(body);
if (txt == null) {
setStatus(Status.CLIENT_ERROR_BAD_REQUEST);
return null;
}
Validations objv = new Validations();
Validation v;
Term term;
try {
term = parseTerm(txt);
} catch (Exception e) {
term = null;
}
if (term == null) v = new Validation(false);
else v = new Validation(true);
objv.addTermValidation(v);
return objv.json();
}
}
| {'content_hash': 'b0d5c5116ee6dfcadfef0164c6a44d05', 'timestamp': '', 'source': 'github', 'line_count': 49, 'max_line_length': 66, 'avg_line_length': 28.979591836734695, 'alnum_prop': 0.6816901408450704, 'repo_name': 'OpenBEL/rest-api', 'id': 'e47088398a7e465196751f8c7b27987701bd0638', 'size': '2029', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'java/src/org/openbel/rest/common/TermValidater.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Clojure', 'bytes': '8141'}, {'name': 'Java', 'bytes': '135845'}, {'name': 'Python', 'bytes': '17834'}, {'name': 'Shell', 'bytes': '10716'}]} |
import os
import smtplib
from validate_email import validate_email
from logger import Logger
class Notification:
"""
Interface that allows user to send notifications using email protocols.
"""
def __init__(self, email_server="127.0.0.1", email_port=587,
email_username="", email_pwd=""):
"""
Set up connection information and authentication tokens to allow user to
access smtp server.
:param email_server: IP Address of SMTP server for sending mail.
:type email_server: string
:param email_port: Port to use to send email
:type email_port: int
:param email_username: Authentication username for SMTP server.
:type email_username: string
:param email_pwd: Authentication username for SMTP server.
:type email_pwd: string
"""
self.email_port = email_port
self.email_server = email_server
self.gmail_user = email_username
self.gmail_pwd = email_pwd
self.logger = Logger()
def build_email(
self,
subject="Notification from Vulnerability",
message="",
source="",
destination=""):
"""
Creates an email notification object from arguments. The email is
constructed using python MIME object types.
:param subject: Subject line of the email.
:type subject: string
:param message: Message body of the email.
:type message: string
:param source: Email address message is sent from.
:type source: string
:param destination: Email address to send message to.
:type destination: string
:returns: MIMEText -- Constructed MIMETextobject with email information
"""
email = MIMEText(text=messgae)
print(email)
def send_notification(self, message="", recipient=""):
"""
Sends a notifiction message to email address specified by recipient.
:param message: Notification message to send
:type message: string
:param recipient: Email address of the recipient
:type recipient: string
:returns: bool -- True if the message was successfuly sent. False otherwise.
"""
TO = recipient
SUBJECT = "Notification from Vulnerability"
TEXT = message
server = smtplib.SMTP(self.email_server, self.email_port)
# Verify that these things are necessary.
server.starttls()
server.ehlo()
# : a login attemt by server
server.login(self.gmail_user, self.gmail_pwd)
BODY = '\r\n'.join(['To: %s' % TO,
'From: %s' % self.gmail_user,
'Subject: %s' % SUBJECT,
'', TEXT])
server.sendmail(self.gmail_user, [TO], BODY)
# NEW IMPLEMENTATION
# email = self.build_email(message=message, soruce=self.gmail_user, destination=recipient)
# server.sendmail(email)
print ('email sent')
server.close()
return True
def notify_all(self, message, recipients):
"""
Sends the message to every email address on the recipient list.
:param message: Notification message to send
:type message: string
:param recipients: List of emails to send notification message
:type recipients: List of strings
:returns: bool -- True if the message was successfuly sent to all
recipients. Otherwise False
"""
success = True
for recipient in recipients:
if not self.send_notification(message, recipient):
success = False
return success
if __name__ == "__main__":
notify = Notifier()
"""
gmail = "smtp.gmail.com"
notification_sender = Notification(
email_server='localhost',
email_port=587,
email_username="",
email_pwd="")
message = "Message I want to send"
source = "Who I want to send the message to. Most likely an email address??"
#notification_sender.send_notification("Hi", '[email protected]')
recipients = []
recipients.append("[email protected]")
recipients.append("[email protected]")
"""
| {'content_hash': 'ef48c687eb11e1ccf6d05d48dbf665c1', 'timestamp': '', 'source': 'github', 'line_count': 131, 'max_line_length': 98, 'avg_line_length': 32.61068702290076, 'alnum_prop': 0.6058052434456929, 'repo_name': 'mikefeneley/chaos-monitor', 'id': '3ddcdf352668b19437e995a215a08be798bbd1d9', 'size': '4272', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/notification.py', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Makefile', 'bytes': '716'}, {'name': 'Python', 'bytes': '86296'}]} |
package com.netflix.spinnaker.orca.igor.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.netflix.spinnaker.kork.artifacts.model.ExpectedArtifact;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import lombok.Getter;
@Getter
public class CIStageDefinition implements RetryableStageDefinition {
private final String master;
private final String job;
private final String propertyFile;
private final Integer buildNumber;
private final boolean waitForCompletion;
private final List<ExpectedArtifact> expectedArtifacts;
private final int consecutiveErrors;
// There does not seem to be a way to auto-generate a constructor using our current version of
// Lombok (1.16.20) that
// Jackson can use to deserialize.
public CIStageDefinition(
@JsonProperty("master") String master,
@JsonProperty("job") String job,
@JsonProperty("property") String propertyFile,
@JsonProperty("buildNumber") Integer buildNumber,
@JsonProperty("waitForCompletion") Boolean waitForCompletion,
@JsonProperty("expectedArtifacts") List<ExpectedArtifact> expectedArtifacts,
@JsonProperty("consecutiveErrors") Integer consecutiveErrors) {
this.master = master;
this.job = job;
this.propertyFile = propertyFile;
this.buildNumber = buildNumber;
this.waitForCompletion = Optional.ofNullable(waitForCompletion).orElse(true);
this.expectedArtifacts =
Collections.unmodifiableList(
Optional.ofNullable(expectedArtifacts).orElse(Collections.emptyList()));
this.consecutiveErrors = Optional.ofNullable(consecutiveErrors).orElse(0);
}
}
| {'content_hash': '464ed053a3e534875181aa97c71ce1b7', 'timestamp': '', 'source': 'github', 'line_count': 43, 'max_line_length': 96, 'avg_line_length': 38.7906976744186, 'alnum_prop': 0.7667865707434053, 'repo_name': 'duftler/orca', 'id': 'b0e984d15dbbfa1cba01d1754db644f463d5d256', 'size': '2260', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'orca-igor/src/main/groovy/com/netflix/spinnaker/orca/igor/model/CIStageDefinition.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Groovy', 'bytes': '2888930'}, {'name': 'HTML', 'bytes': '257'}, {'name': 'Java', 'bytes': '1839730'}, {'name': 'Kotlin', 'bytes': '978079'}, {'name': 'Shell', 'bytes': '2672'}, {'name': 'TSQL', 'bytes': '388'}]} |
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<!--NewPage-->
<HTML>
<HEAD>
<!-- Generated by javadoc (build 1.6.0_20) on Thu Feb 24 01:10:11 PST 2011 -->
<TITLE>
Uses of Class org.apache.hadoop.contrib.failmon.Anonymizer (Hadoop 0.20.2-cdh3u0-SNAPSHOT API)
</TITLE>
<META NAME="date" CONTENT="2011-02-24">
<LINK REL ="stylesheet" TYPE="text/css" HREF="../../../../../../stylesheet.css" TITLE="Style">
<SCRIPT type="text/javascript">
function windowTitle()
{
if (location.href.indexOf('is-external=true') == -1) {
parent.document.title="Uses of Class org.apache.hadoop.contrib.failmon.Anonymizer (Hadoop 0.20.2-cdh3u0-SNAPSHOT API)";
}
}
</SCRIPT>
<NOSCRIPT>
</NOSCRIPT>
</HEAD>
<BODY BGCOLOR="white" onload="windowTitle();">
<HR>
<!-- ========= START OF TOP NAVBAR ======= -->
<A NAME="navbar_top"><!-- --></A>
<A HREF="#skip-navbar_top" title="Skip navigation links"></A>
<TABLE BORDER="0" WIDTH="100%" CELLPADDING="1" CELLSPACING="0" SUMMARY="">
<TR>
<TD COLSPAN=2 BGCOLOR="#EEEEFF" CLASS="NavBarCell1">
<A NAME="navbar_top_firstrow"><!-- --></A>
<TABLE BORDER="0" CELLPADDING="0" CELLSPACING="3" SUMMARY="">
<TR ALIGN="center" VALIGN="top">
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../../overview-summary.html"><FONT CLASS="NavBarFont1"><B>Overview</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../package-summary.html"><FONT CLASS="NavBarFont1"><B>Package</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../../org/apache/hadoop/contrib/failmon/Anonymizer.html" title="class in org.apache.hadoop.contrib.failmon"><FONT CLASS="NavBarFont1"><B>Class</B></FONT></A> </TD>
<TD BGCOLOR="#FFFFFF" CLASS="NavBarCell1Rev"> <FONT CLASS="NavBarFont1Rev"><B>Use</B></FONT> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../package-tree.html"><FONT CLASS="NavBarFont1"><B>Tree</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../../deprecated-list.html"><FONT CLASS="NavBarFont1"><B>Deprecated</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../../index-all.html"><FONT CLASS="NavBarFont1"><B>Index</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../../help-doc.html"><FONT CLASS="NavBarFont1"><B>Help</B></FONT></A> </TD>
</TR>
</TABLE>
</TD>
<TD ALIGN="right" VALIGN="top" ROWSPAN=3><EM>
</EM>
</TD>
</TR>
<TR>
<TD BGCOLOR="white" CLASS="NavBarCell2"><FONT SIZE="-2">
PREV
NEXT</FONT></TD>
<TD BGCOLOR="white" CLASS="NavBarCell2"><FONT SIZE="-2">
<A HREF="../../../../../../index.html?org/apache/hadoop/contrib/failmon//class-useAnonymizer.html" target="_top"><B>FRAMES</B></A>
<A HREF="Anonymizer.html" target="_top"><B>NO FRAMES</B></A>
<SCRIPT type="text/javascript">
<!--
if(window==top) {
document.writeln('<A HREF="../../../../../../allclasses-noframe.html"><B>All Classes</B></A>');
}
//-->
</SCRIPT>
<NOSCRIPT>
<A HREF="../../../../../../allclasses-noframe.html"><B>All Classes</B></A>
</NOSCRIPT>
</FONT></TD>
</TR>
</TABLE>
<A NAME="skip-navbar_top"></A>
<!-- ========= END OF TOP NAVBAR ========= -->
<HR>
<CENTER>
<H2>
<B>Uses of Class<br>org.apache.hadoop.contrib.failmon.Anonymizer</B></H2>
</CENTER>
No usage of org.apache.hadoop.contrib.failmon.Anonymizer
<P>
<HR>
<!-- ======= START OF BOTTOM NAVBAR ====== -->
<A NAME="navbar_bottom"><!-- --></A>
<A HREF="#skip-navbar_bottom" title="Skip navigation links"></A>
<TABLE BORDER="0" WIDTH="100%" CELLPADDING="1" CELLSPACING="0" SUMMARY="">
<TR>
<TD COLSPAN=2 BGCOLOR="#EEEEFF" CLASS="NavBarCell1">
<A NAME="navbar_bottom_firstrow"><!-- --></A>
<TABLE BORDER="0" CELLPADDING="0" CELLSPACING="3" SUMMARY="">
<TR ALIGN="center" VALIGN="top">
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../../overview-summary.html"><FONT CLASS="NavBarFont1"><B>Overview</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../package-summary.html"><FONT CLASS="NavBarFont1"><B>Package</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../../org/apache/hadoop/contrib/failmon/Anonymizer.html" title="class in org.apache.hadoop.contrib.failmon"><FONT CLASS="NavBarFont1"><B>Class</B></FONT></A> </TD>
<TD BGCOLOR="#FFFFFF" CLASS="NavBarCell1Rev"> <FONT CLASS="NavBarFont1Rev"><B>Use</B></FONT> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../package-tree.html"><FONT CLASS="NavBarFont1"><B>Tree</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../../deprecated-list.html"><FONT CLASS="NavBarFont1"><B>Deprecated</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../../index-all.html"><FONT CLASS="NavBarFont1"><B>Index</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../../help-doc.html"><FONT CLASS="NavBarFont1"><B>Help</B></FONT></A> </TD>
</TR>
</TABLE>
</TD>
<TD ALIGN="right" VALIGN="top" ROWSPAN=3><EM>
</EM>
</TD>
</TR>
<TR>
<TD BGCOLOR="white" CLASS="NavBarCell2"><FONT SIZE="-2">
PREV
NEXT</FONT></TD>
<TD BGCOLOR="white" CLASS="NavBarCell2"><FONT SIZE="-2">
<A HREF="../../../../../../index.html?org/apache/hadoop/contrib/failmon//class-useAnonymizer.html" target="_top"><B>FRAMES</B></A>
<A HREF="Anonymizer.html" target="_top"><B>NO FRAMES</B></A>
<SCRIPT type="text/javascript">
<!--
if(window==top) {
document.writeln('<A HREF="../../../../../../allclasses-noframe.html"><B>All Classes</B></A>');
}
//-->
</SCRIPT>
<NOSCRIPT>
<A HREF="../../../../../../allclasses-noframe.html"><B>All Classes</B></A>
</NOSCRIPT>
</FONT></TD>
</TR>
</TABLE>
<A NAME="skip-navbar_bottom"></A>
<!-- ======== END OF BOTTOM NAVBAR ======= -->
<HR>
Copyright © 2009 The Apache Software Foundation
</BODY>
</HTML>
| {'content_hash': '0621508301bb6cde7d723fa94bb267b0', 'timestamp': '', 'source': 'github', 'line_count': 144, 'max_line_length': 237, 'avg_line_length': 42.63194444444444, 'alnum_prop': 0.6149209969050334, 'repo_name': 'simplegeo/hadoop', 'id': 'b4484963eada852d076b69d94d757c58c8422c51', 'size': '6139', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'docs/api/org/apache/hadoop/contrib/failmon/class-use/Anonymizer.html', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'C', 'bytes': '410770'}, {'name': 'C++', 'bytes': '387188'}, {'name': 'Java', 'bytes': '14645413'}, {'name': 'JavaScript', 'bytes': '112012'}, {'name': 'Objective-C', 'bytes': '112020'}, {'name': 'PHP', 'bytes': '152555'}, {'name': 'Perl', 'bytes': '149888'}, {'name': 'Python', 'bytes': '1162926'}, {'name': 'Ruby', 'bytes': '28485'}, {'name': 'Shell', 'bytes': '1625326'}, {'name': 'Smalltalk', 'bytes': '56562'}]} |
function showPic(whichpic){
// 获取超链接属性href ,只是指链接图片的属性
var source = whichpic.getAttribute("href");
// 或者占位图片的ID
var placeholder = document.getElementById('placeholder');
// 替换占位图片的属性,达到切换图片显示效果
placeholder.setAttribute("src",source);
// 获取图片标题属性
var titletext = whichpic.getAttribute("title");
//获取显示文档标题的段落ID
var description_title = document.getElementById("description_title");
//把标题赋值给段落从而达到替换显示
description_title.firstChild.nodeValue = titletext;
// 获取图片alt属性
var titlecontent = whichpic.getAttribute("alt");
//获取显示文档标题的段落ID
var description_content = document.getElementById("description_content");
//把标题赋值给段落从而达到替换显示
description_content.firstChild.nodeValue = titlecontent;
}
| {'content_hash': '87feaf23e418db081cc69fc141155565', 'timestamp': '', 'source': 'github', 'line_count': 29, 'max_line_length': 74, 'avg_line_length': 24.413793103448278, 'alnum_prop': 0.769774011299435, 'repo_name': 'crperlin/Study', 'id': 'eff029abdaa637f36ab450b1916d7a6cc39171a1', 'size': '930', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'Book/JavaScript+DOM编程艺术/js/showPic.js', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '38679'}, {'name': 'HTML', 'bytes': '1227440'}, {'name': 'JavaScript', 'bytes': '627466'}]} |
/*
Given an input string (s) and a pattern (p), implement wildcard pattern matching with support for '?' and '*' where:
'?' Matches any single character.
'*' Matches any sequence of characters (including the empty sequence).
The matching should cover the entire input string (not partial).
Example 1:
Input: s = "aa", p = "a"
Output: false
Explanation: "a" does not match the entire string "aa".
Example 2:
Input: s = "aa", p = "*"
Output: true
Explanation: '*' matches any sequence.
Example 3:
Input: s = "cb", p = "?a"
Output: false
Explanation: '?' matches 'c', but the second letter is 'a', which does not match 'b'.
Example 4:
Input: s = "adceb", p = "*a*b"
Output: true
Explanation: The first '*' matches the empty sequence, while the second '*' matches the substring "dce".
Example 5:
Input: s = "acdcb", p = "a*c?b"
Output: false
Constraints:
0 <= s.length, p.length <= 2000
s contains only lowercase English letters.
p contains only lowercase English letters, '?' or '*'.
*/
public class Solution {
public boolean isMatch(String s, String p) {
boolean[][] match=new boolean[s.length()+1][p.length()+1];
match[s.length()][p.length()]=true;
for(int i=p.length()-1;i>=0;i--){
if(p.charAt(i)!='*')
break;
else
match[s.length()][i]=true;
}
for(int i=s.length()-1;i>=0;i--){
for(int j=p.length()-1;j>=0;j--){
if(s.charAt(i)==p.charAt(j)||p.charAt(j)=='?')
match[i][j]=match[i+1][j+1];
else if(p.charAt(j)=='*')
match[i][j]=match[i+1][j]||match[i][j+1];
else
match[i][j]=false;
}
}
return match[0][0];
}
} | {'content_hash': '9eb49539f36ab3e49e9a80b51b9dc532', 'timestamp': '', 'source': 'github', 'line_count': 72, 'max_line_length': 116, 'avg_line_length': 24.84722222222222, 'alnum_prop': 0.553381777529346, 'repo_name': 'franklingu/leetcode-solutions', 'id': 'da152b0ad2c064120b74b12199f3e58c3bc6ea40', 'size': '1794', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'questions/wildcard-matching/Solution.java', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'C++', 'bytes': '8919'}, {'name': 'Java', 'bytes': '173033'}, {'name': 'Python', 'bytes': '996874'}, {'name': 'Shell', 'bytes': '2559'}]} |
using Avalonia;
namespace AvaloniaEdit.Demo
{
class Program
{
// This method is needed for IDE previewer infrastructure
public static AppBuilder BuildAvaloniaApp()
=> AppBuilder.Configure<App>().UsePlatformDetect();
// The entry point. Things aren't ready yet
public static int Main(string[] args)
=> BuildAvaloniaApp().StartWithClassicDesktopLifetime(args);
}
}
| {'content_hash': '609d9b1428edcf3eadc2b6124d577da9', 'timestamp': '', 'source': 'github', 'line_count': 15, 'max_line_length': 70, 'avg_line_length': 28.666666666666668, 'alnum_prop': 0.6651162790697674, 'repo_name': 'AvaloniaUI/AvaloniaEdit', 'id': '6cf5095b1cbbb1b46e858abec3937c35956d9dc9', 'size': '432', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/AvaloniaEdit.Demo/Program.cs', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'C', 'bytes': '2321'}, {'name': 'C#', 'bytes': '1873518'}, {'name': 'C++', 'bytes': '1943'}, {'name': 'CSS', 'bytes': '524'}, {'name': 'CoffeeScript', 'bytes': '338'}, {'name': 'Dart', 'bytes': '1538'}, {'name': 'Dockerfile', 'bytes': '609'}, {'name': 'Go', 'bytes': '1125'}, {'name': 'Groovy', 'bytes': '1763'}, {'name': 'HLSL', 'bytes': '6330'}, {'name': 'HTML', 'bytes': '792'}, {'name': 'Handlebars', 'bytes': '336'}, {'name': 'Java', 'bytes': '1658'}, {'name': 'JavaScript', 'bytes': '2406'}, {'name': 'Julia', 'bytes': '977'}, {'name': 'Less', 'bytes': '191'}, {'name': 'Lua', 'bytes': '1176'}, {'name': 'Makefile', 'bytes': '2199'}, {'name': 'PHP', 'bytes': '750'}, {'name': 'Pascal', 'bytes': '198'}, {'name': 'Perl', 'bytes': '972'}, {'name': 'Pug', 'bytes': '150'}, {'name': 'R', 'bytes': '265'}, {'name': 'Roff', 'bytes': '843'}, {'name': 'Ruby', 'bytes': '531'}, {'name': 'SCSS', 'bytes': '492'}, {'name': 'Shell', 'bytes': '915'}, {'name': 'Swift', 'bytes': '493'}, {'name': 'TeX', 'bytes': '1794'}, {'name': 'TypeScript', 'bytes': '2197'}, {'name': 'XSLT', 'bytes': '430'}]} |
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.devinci.lib.common"
>
<application android:fullBackupContent="false"/>
</manifest>
| {'content_hash': '8e787ae2f9f3fb8a81bb88e0f7e35b9e', 'timestamp': '', 'source': 'github', 'line_count': 6, 'max_line_length': 68, 'avg_line_length': 29.333333333333332, 'alnum_prop': 0.7272727272727273, 'repo_name': 'devinciltd/lib', 'id': 'fb989153aa4e2fc6484447d9f2f359fbf5158a93', 'size': '176', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'lib-android-common/src/main/AndroidManifest.xml', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Java', 'bytes': '80421'}]} |
title: How can I tell people to get to the point?
link: http://workplace.stackexchange.com/questions/9283/
tags: workplace, meeting
---
Asking questions orients the meeting, use it.
Asking for clarification is not stupid.
| {'content_hash': 'e967167835627f22aa9790bd582bad89', 'timestamp': '', 'source': 'github', 'line_count': 8, 'max_line_length': 56, 'avg_line_length': 28.0, 'alnum_prop': 0.7723214285714286, 'repo_name': 'nobe4/tldr', 'id': '50ead751eb78f071e011a56fd190c52ca0b40554', 'size': '229', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': '2016/06/28/get-to-the-point.md', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Shell', 'bytes': '405'}]} |
import {
ChangeDetectionStrategy,
Component,
Directive,
IterableChanges,
IterableDiffer,
IterableDiffers,
SimpleChanges,
TemplateRef,
ViewContainerRef
} from '@angular/core';
import {CdkCellDef} from './cell';
/**
* The row template that can be used by the md-table. Should not be used outside of the
* material library.
*/
export const CDK_ROW_TEMPLATE = `<ng-container cdkCellOutlet></ng-container>`;
/**
* Base class for the CdkHeaderRowDef and CdkRowDef that handles checking their columns inputs
* for changes and notifying the table.
*/
export abstract class BaseRowDef {
/** The columns to be displayed on this row. */
columns: string[];
/** Differ used to check if any changes were made to the columns. */
protected _columnsDiffer: IterableDiffer<any>;
constructor(public template: TemplateRef<any>,
protected _differs: IterableDiffers) { }
ngOnChanges(changes: SimpleChanges): void {
// Create a new columns differ if one does not yet exist. Initialize it based on initial value
// of the columns property.
const columns = changes['columns'].currentValue;
if (!this._columnsDiffer && columns) {
this._columnsDiffer = this._differs.find(columns).create();
this._columnsDiffer.diff(columns);
}
}
/**
* Returns the difference between the current columns and the columns from the last diff, or null
* if there is no difference.
*/
getColumnsDiff(): IterableChanges<any> | null {
return this._columnsDiffer.diff(this.columns);
}
}
/**
* Header row definition for the CDK table.
* Captures the header row's template and other header properties such as the columns to display.
*/
@Directive({
selector: '[cdkHeaderRowDef]',
inputs: ['columns: cdkHeaderRowDef'],
})
export class CdkHeaderRowDef extends BaseRowDef {
constructor(template: TemplateRef<any>, _differs: IterableDiffers) {
super(template, _differs);
}
}
/**
* Data row definition for the CDK table.
* Captures the header row's template and other row properties such as the columns to display.
*/
@Directive({
selector: '[cdkRowDef]',
inputs: ['columns: cdkRowDefColumns'],
})
export class CdkRowDef extends BaseRowDef {
// TODO(andrewseguin): Add an input for providing a switch function to determine
// if this template should be used.
constructor(template: TemplateRef<any>, _differs: IterableDiffers) {
super(template, _differs);
}
}
/** Context provided to the row cells */
export interface CdkCellOutletRowContext<T> {
/** Data for the row that this cell is located within. */
$implicit: T;
/** Index location of the row that this cell is located within. */
index?: number;
/** Length of the number of total rows. */
count?: number;
/** True if this cell is contained in the first row. */
first?: boolean;
/** True if this cell is contained in the last row. */
last?: boolean;
/** True if this cell is contained in a row with an even-numbered index. */
even?: boolean;
/** True if this cell is contained in a row with an odd-numbered index. */
odd?: boolean;
}
/**
* Outlet for rendering cells inside of a row or header row.
* @docs-private
*/
@Directive({selector: '[cdkCellOutlet]'})
export class CdkCellOutlet {
/** The ordered list of cells to render within this outlet's view container */
cells: CdkCellDef[];
/** The data context to be provided to each cell */
context: any;
/**
* Static property containing the latest constructed instance of this class.
* Used by the CDK table when each CdkHeaderRow and CdkRow component is created using
* createEmbeddedView. After one of these components are created, this property will provide
* a handle to provide that component's cells and context. After init, the CdkCellOutlet will
* construct the cells with the provided context.
*/
static mostRecentCellOutlet: CdkCellOutlet;
constructor(public _viewContainer: ViewContainerRef) {
CdkCellOutlet.mostRecentCellOutlet = this;
}
}
/** Header template container that contains the cell outlet. Adds the right class and role. */
@Component({
selector: 'cdk-header-row',
template: CDK_ROW_TEMPLATE,
host: {
'class': 'cdk-header-row',
'role': 'row',
},
changeDetection: ChangeDetectionStrategy.OnPush,
})
export class CdkHeaderRow { }
/** Data row template container that contains the cell outlet. Adds the right class and role. */
@Component({
selector: 'cdk-row',
template: CDK_ROW_TEMPLATE,
host: {
'class': 'cdk-row',
'role': 'row',
},
changeDetection: ChangeDetectionStrategy.OnPush,
})
export class CdkRow { }
| {'content_hash': '96db08814d3c7a7110d81e40b4df9254', 'timestamp': '', 'source': 'github', 'line_count': 157, 'max_line_length': 99, 'avg_line_length': 29.579617834394906, 'alnum_prop': 0.7054263565891473, 'repo_name': 'nkwood/material2', 'id': '91331a49f9e119dfff18e5d0dda753da00d9f4d4', 'size': '4846', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/cdk/table/row.ts', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '225803'}, {'name': 'HTML', 'bytes': '212479'}, {'name': 'JavaScript', 'bytes': '20731'}, {'name': 'Shell', 'bytes': '24307'}, {'name': 'TypeScript', 'bytes': '2082298'}]} |
<?xml version="1.0" encoding="iso-8859-1"?>
<!DOCTYPE html
PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<!--
Classes
-->
<html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en" lang="en">
<head>
<title>Classes</title>
<meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1" />
<link rel="stylesheet" href="rdoc-style.css" type="text/css" />
<base target="docwin" />
</head>
<body>
<div id="index">
<h1 class="section-bar">Classes</h1>
<div id="index-entries">
<a href="classes/ActionController.html">ActionController</a><br />
<a href="classes/CasLogin.html">CasLogin</a><br />
</div>
</div>
</body>
</html> | {'content_hash': '6946789645d68933ec0b655fe18c25ad', 'timestamp': '', 'source': 'github', 'line_count': 27, 'max_line_length': 76, 'avg_line_length': 26.962962962962962, 'alnum_prop': 0.6373626373626373, 'repo_name': 'mkauffman/bblearn-selfservice-example', 'id': '9a919bd77010e0867dc0b18afdc9bcd8e08e0037', 'size': '729', 'binary': False, 'copies': '1', 'ref': 'refs/heads/examplecode', 'path': 'vendor/plugins/cas_login/rdoc/fr_class_index.html', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'JavaScript', 'bytes': '21691'}, {'name': 'Ruby', 'bytes': '160343'}]} |
<?php
class General extends Application {
var $forum_view = 'forum_3';
var $forum_url = "general";
var $forum_model;
function __construct() {
parent::__construct();
$this->forum_model = $this->posts_general;
}
function index() {
//get the view for the General forum
//this view is planned to be settable by a drop-down menu, but as of now, this is just the view used for the General forum
if(null != $this->input->post('layout'))
$this->forum_view = $this->input->post('layout');
$this->data['pagebody'] = $this->forum_view;
//get the title and posts from the Posts_General model
$title = $this->forum_model->get_title();
$posts = $this->forum_model->get_posts();
foreach($posts as &$post) {
$post['actions'] = "<a href=\"/".$this->forum_url."/reply/".$post['postnum']."\">Reply</a> | "
. "<a href=\"/".$this->forum_url."/quote/".$post['postnum']."\">Quote</a>";
}
apply_layout($posts, $this->forum_view);
//set the $title and $posts data (an array) for use in the view
$this->data['title'] = $title;
$this->data['posts'] = $posts;
$this->data['toggle_admin'] = "<a href=\"/".$this->forum_url."/admin\">Enable Admin</a>";
$this->render();
}
function admin() {
//get the view for the Announcements forum
//this view is planned to be settable by a drop-down menu, but as of now, this is just the view used for the Announcements forum
if(null != $this->input->post('layout'))
$this->forum_view = $this->input->post('layout');
$this->data['pagebody'] = $this->forum_view;
//get the title and posts from the Posts_Announcements model
$title = $this->forum_model->get_title();
$posts = $this->forum_model->get_posts();
//'actions' consists of a link to reply, quote ... according to each post.
//append each of these to each of $posts.
foreach($posts as &$post) {
$post['actions'] = "<a href=\"/".$this->forum_url."/reply/".$post['postnum']."/admin\">Reply</a> | "
. "<a href=\"/".$this->forum_url."/quote/".$post['postnum']."/admin\">Quote</a> | "
. "<a href=\"/".$this->forum_url."/edit/".$post['postnum']."/admin\">Edit</a> | "
. "<a href=\"/".$this->forum_url."/delete/".$post['postnum']."/admin\">Delete</a>";
}
apply_layout($posts, $this->forum_view);
//set the $title, $posts, and $actions data (an array) for use in the view
$this->data['title'] = $title;
$this->data['posts'] = $posts;
$this->data['toggle_admin'] = "<a href=\"/".$this->forum_url."\">Disable Admin</a><br>"
. "<a href=\"/avatars\">Manage User Avatars</a>";
$this->render();
}
//create a reply page
function edit($postnum = 0, $switch = "") {
if($postnum == 0) //not a specified postnum
redirect('/'.$this->forum_url);
$this->data['pagebody'] = 'edit';
//get original post
$title = "Edit Post";
$origpost = $this->forum_model->get_post($postnum);
//set the title, username, message, and post data fo this edit
$this->data['title'] = $title;
$this->data['given_username'] = $origpost['username'];
$this->data['messagebox'] = $origpost['message'];
$this->data['submiturl'] = "/".$this->forum_url."/submitedit/".$postnum."/".$switch; //was missing the initial backslash, which led to an error.
$this->data = array_merge($this->data, $origpost);
$this->render();
}
//submit reply
function submitedit($postnum = 0, $switch = "") {
//through post method
//include username, password, post content ..., put it into database, redirect back to original post
//Update a record that is to be edited in the database.
$record = $this->forum_model->get($postnum);
$record->username = $this->input->post('username');
$record->subject = $this->input->post('subject');
$record->message = $this->input->post('message');
//Set the date that the reply was submitted at.
date_default_timezone_set("America/Vancouver");
$record->date = date("M. j/y, g:iA");
//Update the record, as this is an edit.
$this->forum_model->update($record);
//update the position of this in recent posts
//delete the post from recent_posts - with the forum $forum, and postnum $postnum
$this->recent_posts2->delete($this->forum_url, $postnum);
//add it to recent posts list
$recent_rec = $this->recent_posts->create();
$recent_rec->forum = $this->forum_url;
$recent_rec->postnum = $postnum;
$recent_rec->recency = $this->recent_posts->highest()+1;
$this->recent_posts->add($recent_rec);
redirect('/'.$this->forum_url.'/'.$switch);
//Validation for the reply
//cancel if the reply data was not set
//if(!(isset($this->input->post('subject')) && isset($this->input->post('message'))))
// redirect('/announcements');
//$this->posts_announcements->
}
//delete the post with postnum $postnum
function delete($postnum = 0, $switch = "") {
$this->forum_model->delete($postnum); //deletes the post with the key $postnum
//deletes the post from recent_posts as well - with the forum $forum, and postnum $postnum
$this->recent_posts2->delete($this->forum_url, $postnum);
redirect('/'.$this->forum_url.'/'.$switch);
}
//create a reply page
function reply($postnum = 0, $switch = "") {
if($postnum == 0) //not a specified postnum
redirect('/'.$this->forum_url);
$this->data['pagebody'] = 'reply';
//get original post
$title = "Make a Reply";
$origpost = $this->forum_model->get_post($postnum);
//'actions' consists of a link to reply, quote ... according to each post.
//append each of these to each of $posts.
$origpost['actions'] = "<a href=\"/".$this->forum_url."/reply/".$origpost['postnum']."\">Reply</a> | "
. "<a href=\"/".$this->forum_url."/quote/".$origpost['postnum']."\">Quote</a>";
//set the title, username, message, and original post data
$this->data['title'] = $title;
$this->data['given_username'] = "Anonymous"; //was lost from prior commit as well
$this->data['messagebox'] = "";
$this->data['submiturl'] = "/".$this->forum_url."/submitreply/".$postnum."/".$switch; //was missing the initial backslash, which led to an error.
$this->data = array_merge($this->data, $origpost);
$this->render();
}
//submit reply
function submitreply($postnum = 0, $switch = "") {
//through post method
//include username, password, post content ..., put it into database, redirect back to original post
//Create a record that is to be added to the database, as a new reply.
$record = $this->forum_model->create();
$record->postnum = $this->forum_model->highest()+1;
$record->username = $this->input->post('username');
$record->subject = $this->input->post('subject');
$record->message = $this->input->post('message');
//Set the date that the reply was submitted at.
date_default_timezone_set("America/Vancouver");
$record->date = date("M. j/y, g:iA");
//Save the record created for the reply.
$this->forum_model->add($record);
//add it to recent posts list
$recent_rec = $this->recent_posts->create();
$recent_rec->forum = $this->forum_url;
$recent_rec->postnum = $record->postnum;
$recent_rec->recency = $this->recent_posts->highest()+1;
$this->recent_posts->add($recent_rec);
//set to admin, if admin
if($switch == "admin")
redirect('/'.$this->forum_url.'/admin');
redirect('/'.$this->forum_url);
//Validation for the reply
//cancel if the reply data was not set
//if(!(isset($this->input->post('subject')) && isset($this->input->post('message'))))
// redirect('/announcements');
//$this->posts_announcements->
}
//quote a reply; reply with info from the quoted post, in the message area.
function quote($postnum = 0, $switch = "") {
if($postnum == 0) //not a specified postnum
redirect('/'.$this->forum_url);
$this->data['pagebody'] = 'reply';
//get original post
$title = "Quote";
$origpost = $this->forum_model->get_post($postnum);
//'actions' consists of a link to reply, quote ... according to each post.
//append each of these to each of $posts.
$origpost['actions'] = "<a href=\"/".$this->forum_url."/reply/".$origpost['postnum']."\">Reply</a> | "
. "<a href=\"/".$this->forum_url."/quote/".$origpost['postnum']."\">Quote</a>";
//set the title, username, message, and original post data
$this->data['title'] = $title;
$this->data['given_username'] = "Anonymous"; //was lost from prior commit as well
$this->data['messagebox'] = "<p>\r\n".$origpost['username']." wrote:<br>\r\n". $origpost['message']."\r\n</p>\r\n\r\n";
$this->data['submiturl'] = "/".$this->forum_url."/submitreply/".$postnum."/".$switch; //was missing the initial backslash, which led to an error.
$this->data = array_merge($this->data, $origpost);
$this->render();
}
} | {'content_hash': '2df64d9d2bef921adbed6685dd24dd7d', 'timestamp': '', 'source': 'github', 'line_count': 231, 'max_line_length': 153, 'avg_line_length': 43.84848484848485, 'alnum_prop': 0.541514463421858, 'repo_name': 'Subrosian/COMP4711-Subforum', 'id': '03c3aef2b488851d72cf0b2a02e592672f18cf00', 'size': '10129', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'application/controllers/General.php', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'ApacheConf', 'bytes': '375'}, {'name': 'CSS', 'bytes': '7795'}, {'name': 'HTML', 'bytes': '1710'}, {'name': 'PHP', 'bytes': '169095'}]} |
<?php
session_start();
include("../../config/base.inc.php");
include("../../config/login.php");
if($_SESSION[Tipo] == "Administrador"){
$titulo = $_POST['TituloTodos'];
$mensaje = $_POST['MensajeTodos'];
$cabeceras = 'From: PTA';
$consulta = mysql_query("SELECT * FROM Usuarios",$conn) or die ('Error al seleccionar la Base de Datos: '.mysql_error());
while($row = mysql_fetch_assoc($consulta)){
$para = $row['Correo'];
mail($para, $titulo, $mensaje, $cabeceras);
}
sleep(3);
header("Location: ../resultado/exito.php");
}else{
echo "No tiene los suficientes permisos para realizar esta tarea.";
}
?> | {'content_hash': 'e0892fa9b972aa08e489bf1bf6d8a3fa', 'timestamp': '', 'source': 'github', 'line_count': 23, 'max_line_length': 123, 'avg_line_length': 28.0, 'alnum_prop': 0.6211180124223602, 'repo_name': 'IsraelSC/PTA', 'id': '1b01fe75fcb23b9513be0cc57a18a9df023068c4', 'size': '644', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'secciones/correo/procesa_correo_todos.php', 'mode': '33261', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '30837'}, {'name': 'HTML', 'bytes': '4018'}, {'name': 'JavaScript', 'bytes': '28918'}, {'name': 'PHP', 'bytes': '4570814'}]} |
namespace bpt = boost::property_tree;
namespace {
void test_tilejson_fetch() {
char cwd[PATH_MAX];
if (getcwd(&cwd[0], PATH_MAX) == NULL) { throw std::runtime_error("getcwd failed"); }
bpt::ptree conf = avecado::tilejson((boost::format("file://%1%/test/tilejson.json") % cwd).str());
test::assert_equal<int>(conf.get<int>("maskLevel"), 8, "maskLevel");
test::assert_equal<int>(conf.get<int>("maxzoom"), 15, "maxzoom");
test::assert_equal<int>(conf.get<int>("minzoom"), 0, "minzoom");
}
// TODO: this is a pretty awful test, and isn't able to dig any deeper than
// the overzoom, not even to inspect it. might need an upgrade to how the
// built-in HTTP server works so that we can do more with these tests...
void test_tilejson_parse() {
using namespace avecado;
char cwd[PATH_MAX];
if (getcwd(&cwd[0], PATH_MAX) == NULL) { throw std::runtime_error("getcwd failed"); }
bpt::ptree conf = tilejson((boost::format("file://%1%/test/tilejson.json") % cwd).str());
std::unique_ptr<fetcher> f = make_tilejson_fetcher(conf);
fetch::overzoom *o = dynamic_cast<fetch::overzoom *>(f.get());
test::assert_equal<bool>(o != nullptr, true, "is overzoom");
}
// utility function to create TileJSON from Mapnik XML.
std::string tile_json_for_xml(const std::string xml) {
using test::make_map;
using avecado::make_tilejson;
char cwd[PATH_MAX];
if (getcwd(&cwd[0], PATH_MAX) == NULL) { throw std::runtime_error("getcwd failed"); }
mapnik::Map map = make_map(xml, 256, 0, 0, 0);
std::string json = make_tilejson(map, (boost::format("file://%1%/") % cwd).str());
return json;
}
// https://github.com/MapQuest/avecado/issues/54
//
// maxzoom, minzoom & metatile (masklevel, presumably, too) are all
// supposed to be numeric, but are being generated as strings.
//
// the 2.1.0 tilejson spec doesn't specify metatile or masklevel,
// but we'll just assume they're supposed to be integers too.
void test_tilejson_generate_numeric() {
using namespace boost::xpressive;
for (auto &xml : {"test/empty_map_file.xml", "test/tilejson_params.xml"}) {
try {
// this test is kinda half-assed, what with the regex and all...
// but it should work to detect the difference between a JSON
// string and a number.
const std::string json = tile_json_for_xml(xml);
for (auto ¶m : {"metatile", "maskLevel", "minzoom", "maxzoom"}) {
// first, need to check if the key is present at all. if not,
// then it doesn't make sense to check that it's a number.
// NOTE: this is /"${param}" *:/
sregex is_present = as_xpr('"') >> param >> '"' >> *space >> as_xpr(':');
if (regex_search(json.begin(), json.end(), is_present)) {
// if present, we check if the value begins with a valid number
// prefix: an optional minus sign and then digits. this might
// match invalid JSON, but the validity of generated JSON is a
// test we'll have to do elsewhere.
sregex is_number = as_xpr('"') >> param >> '"' >> *space >> as_xpr(':')
>> *space >> !as_xpr('-') >> +digit;
if (!regex_search(json.begin(), json.end(), is_number)) {
throw std::runtime_error(
(boost::format("Parameter \"%1%\" should be a number, but is not "
"in TileJSON: %2%") % param % json).str());
}
}
}
// Some params need to be arrays of integers
for (auto ¶m : {"center", "bounds"}) {
// The same check as above for presense
sregex is_present = as_xpr('"') >> param >> '"' >> *space >> as_xpr(':');
if (regex_search(json.begin(), json.end(), is_present)) {
sregex is_array = as_xpr('"') >> param >> '"' >> *space >> as_xpr(':')
>> *space >> as_xpr('[');
if (!regex_search(json.begin(), json.end(), is_array)) {
throw std::runtime_error(
(boost::format("Parameter \"%1%\" should be an array of numbers, but is not "
"in TileJSON: %2%") % param % json).str());
}
}
}
} catch (const std::exception &e) {
std::throw_with_nested(
std::runtime_error(
(boost::format("while processing XML file \"%1%\"") % xml).str()));
}
}
}
// same as the above test, but we force Mapnik to store strings
// in its parameter list so that we have to convert to numeric
// before outputting to JSON.
void test_tilejson_generate_numeric_force() {
using namespace boost::xpressive;
using test::make_map;
using avecado::make_tilejson;
char cwd[PATH_MAX];
if (getcwd(&cwd[0], PATH_MAX) == NULL) { throw std::runtime_error("getcwd failed"); }
mapnik::Map map = make_map("test/empty_map_file.xml", 256, 0, 0, 0);
map.get_extra_parameters().emplace("maxzoom", mapnik::value_holder(std::string("0")));
std::string json = make_tilejson(map, (boost::format("file://%1%/") % cwd).str());
sregex is_present = as_xpr("\"maxzoom\"") >> *space >> as_xpr(':');
if (!regex_search(json.begin(), json.end(), is_present)) {
throw std::runtime_error(
(boost::format("maxzoom key not in generated JSON: %1%") % json).str());
}
sregex is_number = as_xpr("\"maxzoom\"") >> *space >> as_xpr(':')
>> *space >> !as_xpr('-') >> +digit;
if (!regex_search(json.begin(), json.end(), is_number)) {
throw std::runtime_error(
(boost::format("Parameter \"maxzoom\" should be a number, but is not "
"in TileJSON: %1%") % json).str());
}
}
void test_tilejson_generate_masklevel() {
using test::make_map;
using avecado::make_tilejson;
test::temp_dir tmp;
std::string base_url = (boost::format("file://%1%/") % tmp.path().native()).str();
mapnik::Map map = make_map("test/empty_map_file.xml", 256, 0, 0, 0);
for (int maxzoom = 0; maxzoom < 23; ++maxzoom) {
map.get_extra_parameters().erase("maxzoom");
map.get_extra_parameters().emplace("maxzoom", mapnik::value_integer(maxzoom));
const std::string json = make_tilejson(map, base_url);
const std::string json_file = (tmp.path() / "tile.json").native();
std::ofstream out(json_file);
out.write(json.data(), json.size());
out.close();
bpt::ptree conf = avecado::tilejson((boost::format("%1%tile.json") % base_url).str());
test::assert_equal<mapnik::value_integer>(
map.get_extra_parameters().find("maxzoom")->second.get<mapnik::value_integer>(),
mapnik::value_integer(maxzoom), "maxzoom parameter");
test::assert_equal<size_t>(map.get_extra_parameters().count("maskLevel"), 0,
"maskLevel presence in original parameters");
test::assert_equal<int>(conf.get<int>("maxzoom"), maxzoom, "maxzoom");
test::assert_equal<int>(conf.get<int>("maskLevel"), maxzoom, "maskLevel");
}
}
} // anonymous namespace
int main() {
int tests_failed = 0;
std::cout << "== Testing TileJSON parsing ==" << std::endl << std::endl;
#define RUN_TEST(x) { tests_failed += test::run(#x, &(x)); }
RUN_TEST(test_tilejson_fetch);
RUN_TEST(test_tilejson_parse);
RUN_TEST(test_tilejson_generate_numeric);
RUN_TEST(test_tilejson_generate_numeric_force);
RUN_TEST(test_tilejson_generate_masklevel);
std::cout << " >> Tests failed: " << tests_failed << std::endl << std::endl;
return (tests_failed > 0) ? 1 : 0;
}
| {'content_hash': '857005151da59dd7a3c293594d4534de', 'timestamp': '', 'source': 'github', 'line_count': 185, 'max_line_length': 100, 'avg_line_length': 40.32972972972973, 'alnum_prop': 0.5989813697895724, 'repo_name': 'zerebubuth/avecado', 'id': '0bd36416aab17d2b02af62abac050fc195343654', 'size': '7697', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'test/tilejson.cpp', 'mode': '33188', 'license': 'bsd-2-clause', 'language': [{'name': 'C++', 'bytes': '316885'}, {'name': 'Python', 'bytes': '1859'}, {'name': 'Shell', 'bytes': '73'}]} |
package com.snell.michael.kawaii.string;
import com.snell.michael.kawaii.ComparableMicroType;
public abstract class StringMicroType extends ComparableMicroType<String> implements CharSequence {
public StringMicroType(String value) {
super(value);
}
@Override
public int length() {
return value.length();
}
@Override
public char charAt(int index) {
return value.charAt(index);
}
@Override
public CharSequence subSequence(int start, int end) {
return value.subSequence(start, end);
}
}
| {'content_hash': '2393c525138f85e7c70a6ca96c47d9bd', 'timestamp': '', 'source': 'github', 'line_count': 24, 'max_line_length': 99, 'avg_line_length': 23.541666666666668, 'alnum_prop': 0.6814159292035398, 'repo_name': 'snellm/kawaii', 'id': '10d849506b2b5dab2dde9ce9fdf2cfa3a71e670c', 'size': '565', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/main/java/com/snell/michael/kawaii/string/StringMicroType.java', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Java', 'bytes': '29202'}]} |
#include <arch_locate_protocols.h>
#include <debug.h>
#include <dump_vmm_on_error_if_needed.h>
#include <efi/efi_loaded_image_protocol.h>
#include <efi/efi_simple_file_system_protocol.h>
#include <efi/efi_status.h>
#include <efi/efi_system_table.h>
#include <efi/efi_types.h>
#include <loader_init.h>
#include <platform.h>
#include <serial_init.h>
#include <span_t.h>
#include <start_vmm.h>
#include <start_vmm_args_t.h>
/**
* NOTE:
* - We always return EFI_SUCCESS, even on failure as on some systems,
* returning something else will cause the system to halt.
*/
/** @brief defines the global pointer to the EFI_SYSTEM_TABLE */
EFI_SYSTEM_TABLE *g_st = NULL;
/** @brief defines the global pointer to the EFI_LOADED_IMAGE_PROTOCOL */
EFI_LOADED_IMAGE_PROTOCOL *g_loaded_image_protocol = NULL;
/** @brief defines the global pointer to the EFI_SIMPLE_FILE_SYSTEM_PROTOCOL */
EFI_SIMPLE_FILE_SYSTEM_PROTOCOL *g_simple_file_system_protocol = NULL;
/**
* <!-- description -->
* @brief Returns the size (in bytes) of the provided file
*
* <!-- inputs/outputs -->
* @param file_protocol the file protocol for the file to query
* @param file_size where to return the resulting file size
* @return returns EFI_SUCCESS on success, and a non-EFI_SUCCESS value on
* failure.
*/
EFI_STATUS
get_file_size(EFI_FILE_PROTOCOL *const file_protocol, UINTN *const file_size)
{
EFI_STATUS status = EFI_SUCCESS;
EFI_GUID efi_file_info_guid = EFI_FILE_INFO_ID;
EFI_FILE_INFO *efi_file_info = NULL;
UINTN efi_file_info_size = 0;
/**
* NOTE:
* - We don't know what the size of the EFI_FILE_INFO is. To get that,
* we need to run GetInfo with the size set to 0. It will return the
* size that we need, and then from there we have to allocate a buffer,
* get the file size, and then free this buffer.
*/
status = file_protocol->GetInfo(file_protocol, &efi_file_info_guid, &efi_file_info_size, NULL);
if (status != EFI_BUFFER_TOO_SMALL) {
bferror_x64("GetInfo failed", status);
goto get_info_failed_size;
}
status = g_st->BootServices->AllocatePool(
EfiLoaderData, efi_file_info_size, ((VOID **)&efi_file_info));
if (EFI_ERROR(status)) {
bferror_x64("AllocatePool failed", status);
goto allocate_pool_failed;
}
status = file_protocol->GetInfo(
file_protocol, &efi_file_info_guid, &efi_file_info_size, efi_file_info);
if (EFI_ERROR(status)) {
bferror_x64("GetInfo failed", status);
goto get_info_failed;
}
*file_size = efi_file_info->FileSize;
g_st->BootServices->FreePool(efi_file_info);
return EFI_SUCCESS;
get_info_failed:
g_st->BootServices->FreePool(efi_file_info);
allocate_pool_failed:
get_info_failed_size:
*file_size = 0;
return status;
}
/**
* <!-- description -->
* @brief Allocates a buffer and fills the buffer with the contents of the
* provided file.
*
* <!-- inputs/outputs -->
* @param volume_protocol the file protocol for the boot volume
* @param filename the file to read
* @param file where to store the resulting buffer containing the file
* contents. This buffer must be freed by the caller.
* @return returns EFI_SUCCESS on success, and a non-EFI_SUCCESS value on
* failure.
*/
EFI_STATUS
read_file(
EFI_FILE_PROTOCOL *const volume_protocol, CHAR16 *const filename, struct span_t *const file)
{
EFI_STATUS status = EFI_SUCCESS;
EFI_FILE_PROTOCOL *file_protocol = NULL;
status =
volume_protocol->Open(volume_protocol, &file_protocol, filename, EFI_FILE_MODE_READ, 0);
if (EFI_ERROR(status)) {
bferror_x64("Open failed", status);
goto open_failed;
}
status = get_file_size(file_protocol, &file->size);
if (EFI_ERROR(status)) {
bferror_x64("get_file_size failed", status);
goto get_file_size_failed;
}
status =
g_st->BootServices->AllocatePool(EfiRuntimeServicesData, file->size, (VOID **)&file->addr);
if (EFI_ERROR(status)) {
bferror_x64("AllocatePool failed", status);
goto allocate_pool_failed;
}
status = file_protocol->Read(file_protocol, &file->size, (VOID *)file->addr);
if (EFI_ERROR(status)) {
bferror_x64("Read failed", status);
goto read_failed;
}
file_protocol->Close(file_protocol);
return EFI_SUCCESS;
read_failed:
g_st->BootServices->FreePool((VOID *)file->addr);
file->addr = NULL;
file->size = ((uint64_t)0);
allocate_pool_failed:
get_file_size_failed:
file_protocol->Close(file_protocol);
open_failed:
return status;
}
/**
* <!-- description -->
* @brief Locates all of the protocols that are needed
*
* <!-- inputs/outputs -->
* @return returns EFI_SUCCESS on success, and a non-EFI_SUCCESS value on
* failure.
*/
EFI_STATUS
locate_protocols(EFI_HANDLE ImageHandle)
{
EFI_STATUS status = EFI_SUCCESS;
EFI_GUID efi_loaded_image_protocol_guid = EFI_LOADED_IMAGE_PROTOCOL_GUID;
EFI_GUID efi_simple_file_system_protocol_guid = EFI_SIMPLE_FILE_SYSTEM_PROTOCOL_GUID;
status = arch_locate_protocols();
if (EFI_ERROR(status)) {
bferror_x64("arch_locate_protocols failed", status);
return status;
}
status = g_st->BootServices->HandleProtocol(
ImageHandle, &efi_loaded_image_protocol_guid, (VOID **)&g_loaded_image_protocol);
if (EFI_ERROR(status)) {
bferror_x64("HandleProtocol EFI_LOADED_IMAGE_PROTOCOL failed", status);
return status;
}
status = g_st->BootServices->HandleProtocol(
g_loaded_image_protocol->DeviceHandle,
&efi_simple_file_system_protocol_guid,
(VOID **)&g_simple_file_system_protocol);
if (EFI_ERROR(status)) {
bferror_x64("HandleProtocol EFI_SIMPLE_FILE_SYSTEM_PROTOCOL failed", status);
return status;
}
return EFI_SUCCESS;
}
/**
* <!-- description -->
* @brief Loads the ELF images from the EFI partition and starts the VMM.
*
* <!-- inputs/outputs -->
* @return returns EFI_SUCCESS on success, and a non-EFI_SUCCESS value on
* failure.
*/
EFI_STATUS
load_images_and_start(void)
{
EFI_STATUS status = EFI_SUCCESS;
EFI_FILE_PROTOCOL *volume_protocol = NULL;
struct start_vmm_args_t start_args = {0};
status =
g_simple_file_system_protocol->OpenVolume(g_simple_file_system_protocol, &volume_protocol);
if (EFI_ERROR(status)) {
bferror_x64("OpenVolume failed", status);
return status;
}
status = read_file(volume_protocol, L"bareflank_kernel", &start_args.mk_elf_file);
if (EFI_ERROR(status)) {
bferror_x64("open_kernel failed", status);
return status;
}
status = read_file(volume_protocol, L"bareflank_extension0", &(start_args.ext_elf_files[0]));
if (EFI_ERROR(status)) {
bferror_x64("open_extensions failed", status);
return status;
}
start_args.ver = ((uint64_t)1);
start_args.num_pages_in_page_pool = ((uint32_t)0);
if (start_vmm(&start_args)) {
bferror("start_vmm failed");
return EFI_LOAD_ERROR;
}
return EFI_SUCCESS;
}
void serial_write_hex(uint64_t const val);
/**
* <!-- description -->
* @brief Defines the main EFI entry pointer
*
* <!-- inputs/outputs -->
* @param ImageHandle ignored
* @param SystemTable stores a pointer to the global EFI stytem table
* @return returns EFI_SUCCESS on success, and a non-EFI_SUCCESS value on
* failure.
*/
EFI_STATUS
efi_main(EFI_HANDLE ImageHandle, EFI_SYSTEM_TABLE *SystemTable)
{
EFI_STATUS status = EFI_SUCCESS;
/**
* NOTE:
* - This function needs to be kept pretty simple as if you attempt to
* do too much, you might cause memcpy/memset to be called prior to
* the system table being saved.
*/
g_st = SystemTable;
if (g_st->Hdr.Revision < EFI_1_10_SYSTEM_TABLE_REVISION) {
g_st->ConOut->OutputString(g_st->ConOut, L"EFI version not supported\r\n");
return EFI_SUCCESS;
}
serial_init();
status = locate_protocols(ImageHandle);
if (EFI_ERROR(status)) {
bferror_x64("locate_protocols failed", status);
return EFI_SUCCESS;
}
if (loader_init()) {
bferror("loader_init failed");
return EFI_SUCCESS;
}
status = load_images_and_start();
if (EFI_ERROR(status)) {
bferror_x64("load_images_and_start failed", status);
platform_dump_vmm();
return EFI_SUCCESS;
}
platform_dump_vmm();
g_st->ConOut->OutputString(g_st->ConOut, L"bareflank successfully started\r\n");
return EFI_SUCCESS;
}
| {'content_hash': '8ee49bfb19860179fa820722b18f1c6f', 'timestamp': '', 'source': 'github', 'line_count': 293, 'max_line_length': 99, 'avg_line_length': 29.679180887372013, 'alnum_prop': 0.6479990800367985, 'repo_name': 'Bareflank/hypervisor', 'id': 'c448615d6d00108bbf692b9daf3e7aa115cdfd18', 'size': '9891', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'loader/efi/src/entry.c', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Assembly', 'bytes': '65359'}, {'name': 'C', 'bytes': '128272'}, {'name': 'C++', 'bytes': '4106171'}, {'name': 'CMake', 'bytes': '214959'}, {'name': 'Makefile', 'bytes': '1885'}, {'name': 'Shell', 'bytes': '19153'}]} |
package wh1spr.olympians.apollo.music;
import com.sedmelluq.discord.lavaplayer.player.AudioPlayer;
import com.sedmelluq.discord.lavaplayer.track.playback.AudioFrame;
import net.dv8tion.jda.core.audio.AudioSendHandler;
public class AudioSender implements AudioSendHandler {
private AudioPlayer audioPlayer;
private AudioFrame lastFrame;
public AudioSender(AudioPlayer player) {
this.audioPlayer = player;
}
@Override
public boolean canProvide() {
if (lastFrame == null) lastFrame = audioPlayer.provide();
return lastFrame != null;
}
@Override
public byte[] provide20MsAudio() {
if (lastFrame == null) return lastFrame.data;
byte[] data = lastFrame!=null ? lastFrame.data : null;
lastFrame = null;
return data;
}
@Override
public boolean isOpus() {
return true;
}
}
| {'content_hash': '8b72cc72046d4ed67b9a2dc4bf9025db', 'timestamp': '', 'source': 'github', 'line_count': 36, 'max_line_length': 66, 'avg_line_length': 22.5, 'alnum_prop': 0.7444444444444445, 'repo_name': 'Wh1spr/Olympians', 'id': 'cfc8dc830f92a520ef1bef0caedd7394ed0aa95b', 'size': '810', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/main/java/wh1spr/olympians/apollo/music/AudioSender.java', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Java', 'bytes': '76778'}]} |
using System;
using System.Globalization;
using System.Linq;
using System.Security.Claims;
using System.Threading.Tasks;
using System.Web;
using System.Web.Mvc;
using Microsoft.AspNet.Identity;
using Microsoft.AspNet.Identity.Owin;
using Microsoft.Owin.Security;
using Model.Models;
namespace Model.Controllers
{
[Authorize]
public class AccountController : Controller
{
private ApplicationSignInManager _signInManager;
private ApplicationUserManager _userManager;
public AccountController()
{
}
public AccountController(ApplicationUserManager userManager, ApplicationSignInManager signInManager )
{
UserManager = userManager;
SignInManager = signInManager;
}
public ApplicationSignInManager SignInManager
{
get
{
return _signInManager ?? HttpContext.GetOwinContext().Get<ApplicationSignInManager>();
}
private set
{
_signInManager = value;
}
}
public ApplicationUserManager UserManager
{
get
{
return _userManager ?? HttpContext.GetOwinContext().GetUserManager<ApplicationUserManager>();
}
private set
{
_userManager = value;
}
}
//
// GET: /Account/Login
[AllowAnonymous]
public ActionResult Login(string returnUrl)
{
ViewBag.ReturnUrl = returnUrl;
return View();
}
//
// POST: /Account/Login
[HttpPost]
[AllowAnonymous]
[ValidateAntiForgeryToken]
public async Task<ActionResult> Login(LoginViewModel model, string returnUrl)
{
if (!ModelState.IsValid)
{
return View(model);
}
// This doesn't count login failures towards account lockout
// To enable password failures to trigger account lockout, change to shouldLockout: true
var result = await SignInManager.PasswordSignInAsync(model.Email, model.Password, model.RememberMe, shouldLockout: false);
switch (result)
{
case SignInStatus.Success:
return RedirectToLocal(returnUrl);
case SignInStatus.LockedOut:
return View("Lockout");
case SignInStatus.RequiresVerification:
return RedirectToAction("SendCode", new { ReturnUrl = returnUrl, RememberMe = model.RememberMe });
case SignInStatus.Failure:
default:
ModelState.AddModelError("", "Invalid login attempt.");
return View(model);
}
}
//
// GET: /Account/VerifyCode
[AllowAnonymous]
public async Task<ActionResult> VerifyCode(string provider, string returnUrl, bool rememberMe)
{
// Require that the user has already logged in via username/password or external login
if (!await SignInManager.HasBeenVerifiedAsync())
{
return View("Error");
}
return View(new VerifyCodeViewModel { Provider = provider, ReturnUrl = returnUrl, RememberMe = rememberMe });
}
//
// POST: /Account/VerifyCode
[HttpPost]
[AllowAnonymous]
[ValidateAntiForgeryToken]
public async Task<ActionResult> VerifyCode(VerifyCodeViewModel model)
{
if (!ModelState.IsValid)
{
return View(model);
}
// The following code protects for brute force attacks against the two factor codes.
// If a user enters incorrect codes for a specified amount of time then the user account
// will be locked out for a specified amount of time.
// You can configure the account lockout settings in IdentityConfig
var result = await SignInManager.TwoFactorSignInAsync(model.Provider, model.Code, isPersistent: model.RememberMe, rememberBrowser: model.RememberBrowser);
switch (result)
{
case SignInStatus.Success:
return RedirectToLocal(model.ReturnUrl);
case SignInStatus.LockedOut:
return View("Lockout");
case SignInStatus.Failure:
default:
ModelState.AddModelError("", "Invalid code.");
return View(model);
}
}
//
// GET: /Account/Register
[AllowAnonymous]
public ActionResult Register()
{
return View();
}
//
// POST: /Account/Register
[HttpPost]
[AllowAnonymous]
[ValidateAntiForgeryToken]
public async Task<ActionResult> Register(RegisterViewModel model)
{
if (ModelState.IsValid)
{
var user = new ApplicationUser { UserName = model.Email, Email = model.Email };
var result = await UserManager.CreateAsync(user, model.Password);
if (result.Succeeded)
{
await SignInManager.SignInAsync(user, isPersistent:false, rememberBrowser:false);
// For more information on how to enable account confirmation and password reset please visit http://go.microsoft.com/fwlink/?LinkID=320771
// Send an email with this link
// string code = await UserManager.GenerateEmailConfirmationTokenAsync(user.Id);
// var callbackUrl = Url.Action("ConfirmEmail", "Account", new { userId = user.Id, code = code }, protocol: Request.Url.Scheme);
// await UserManager.SendEmailAsync(user.Id, "Confirm your account", "Please confirm your account by clicking <a href=\"" + callbackUrl + "\">here</a>");
return RedirectToAction("Index", "Home");
}
AddErrors(result);
}
// If we got this far, something failed, redisplay form
return View(model);
}
//
// GET: /Account/ConfirmEmail
[AllowAnonymous]
public async Task<ActionResult> ConfirmEmail(string userId, string code)
{
if (userId == null || code == null)
{
return View("Error");
}
var result = await UserManager.ConfirmEmailAsync(userId, code);
return View(result.Succeeded ? "ConfirmEmail" : "Error");
}
//
// GET: /Account/ForgotPassword
[AllowAnonymous]
public ActionResult ForgotPassword()
{
return View();
}
//
// POST: /Account/ForgotPassword
[HttpPost]
[AllowAnonymous]
[ValidateAntiForgeryToken]
public async Task<ActionResult> ForgotPassword(ForgotPasswordViewModel model)
{
if (ModelState.IsValid)
{
var user = await UserManager.FindByNameAsync(model.Email);
if (user == null || !(await UserManager.IsEmailConfirmedAsync(user.Id)))
{
// Don't reveal that the user does not exist or is not confirmed
return View("ForgotPasswordConfirmation");
}
// For more information on how to enable account confirmation and password reset please visit http://go.microsoft.com/fwlink/?LinkID=320771
// Send an email with this link
// string code = await UserManager.GeneratePasswordResetTokenAsync(user.Id);
// var callbackUrl = Url.Action("ResetPassword", "Account", new { userId = user.Id, code = code }, protocol: Request.Url.Scheme);
// await UserManager.SendEmailAsync(user.Id, "Reset Password", "Please reset your password by clicking <a href=\"" + callbackUrl + "\">here</a>");
// return RedirectToAction("ForgotPasswordConfirmation", "Account");
}
// If we got this far, something failed, redisplay form
return View(model);
}
//
// GET: /Account/ForgotPasswordConfirmation
[AllowAnonymous]
public ActionResult ForgotPasswordConfirmation()
{
return View();
}
//
// GET: /Account/ResetPassword
[AllowAnonymous]
public ActionResult ResetPassword(string code)
{
return code == null ? View("Error") : View();
}
//
// POST: /Account/ResetPassword
[HttpPost]
[AllowAnonymous]
[ValidateAntiForgeryToken]
public async Task<ActionResult> ResetPassword(ResetPasswordViewModel model)
{
if (!ModelState.IsValid)
{
return View(model);
}
var user = await UserManager.FindByNameAsync(model.Email);
if (user == null)
{
// Don't reveal that the user does not exist
return RedirectToAction("ResetPasswordConfirmation", "Account");
}
var result = await UserManager.ResetPasswordAsync(user.Id, model.Code, model.Password);
if (result.Succeeded)
{
return RedirectToAction("ResetPasswordConfirmation", "Account");
}
AddErrors(result);
return View();
}
//
// GET: /Account/ResetPasswordConfirmation
[AllowAnonymous]
public ActionResult ResetPasswordConfirmation()
{
return View();
}
//
// POST: /Account/ExternalLogin
[HttpPost]
[AllowAnonymous]
[ValidateAntiForgeryToken]
public ActionResult ExternalLogin(string provider, string returnUrl)
{
// Request a redirect to the external login provider
return new ChallengeResult(provider, Url.Action("ExternalLoginCallback", "Account", new { ReturnUrl = returnUrl }));
}
//
// GET: /Account/SendCode
[AllowAnonymous]
public async Task<ActionResult> SendCode(string returnUrl, bool rememberMe)
{
var userId = await SignInManager.GetVerifiedUserIdAsync();
if (userId == null)
{
return View("Error");
}
var userFactors = await UserManager.GetValidTwoFactorProvidersAsync(userId);
var factorOptions = userFactors.Select(purpose => new SelectListItem { Text = purpose, Value = purpose }).ToList();
return View(new SendCodeViewModel { Providers = factorOptions, ReturnUrl = returnUrl, RememberMe = rememberMe });
}
//
// POST: /Account/SendCode
[HttpPost]
[AllowAnonymous]
[ValidateAntiForgeryToken]
public async Task<ActionResult> SendCode(SendCodeViewModel model)
{
if (!ModelState.IsValid)
{
return View();
}
// Generate the token and send it
if (!await SignInManager.SendTwoFactorCodeAsync(model.SelectedProvider))
{
return View("Error");
}
return RedirectToAction("VerifyCode", new { Provider = model.SelectedProvider, ReturnUrl = model.ReturnUrl, RememberMe = model.RememberMe });
}
//
// GET: /Account/ExternalLoginCallback
[AllowAnonymous]
public async Task<ActionResult> ExternalLoginCallback(string returnUrl)
{
var loginInfo = await AuthenticationManager.GetExternalLoginInfoAsync();
if (loginInfo == null)
{
return RedirectToAction("Login");
}
// Sign in the user with this external login provider if the user already has a login
var result = await SignInManager.ExternalSignInAsync(loginInfo, isPersistent: false);
switch (result)
{
case SignInStatus.Success:
return RedirectToLocal(returnUrl);
case SignInStatus.LockedOut:
return View("Lockout");
case SignInStatus.RequiresVerification:
return RedirectToAction("SendCode", new { ReturnUrl = returnUrl, RememberMe = false });
case SignInStatus.Failure:
default:
// If the user does not have an account, then prompt the user to create an account
ViewBag.ReturnUrl = returnUrl;
ViewBag.LoginProvider = loginInfo.Login.LoginProvider;
return View("ExternalLoginConfirmation", new ExternalLoginConfirmationViewModel { Email = loginInfo.Email });
}
}
//
// POST: /Account/ExternalLoginConfirmation
[HttpPost]
[AllowAnonymous]
[ValidateAntiForgeryToken]
public async Task<ActionResult> ExternalLoginConfirmation(ExternalLoginConfirmationViewModel model, string returnUrl)
{
if (User.Identity.IsAuthenticated)
{
return RedirectToAction("Index", "Manage");
}
if (ModelState.IsValid)
{
// Get the information about the user from the external login provider
var info = await AuthenticationManager.GetExternalLoginInfoAsync();
if (info == null)
{
return View("ExternalLoginFailure");
}
var user = new ApplicationUser { UserName = model.Email, Email = model.Email };
var result = await UserManager.CreateAsync(user);
if (result.Succeeded)
{
result = await UserManager.AddLoginAsync(user.Id, info.Login);
if (result.Succeeded)
{
await SignInManager.SignInAsync(user, isPersistent: false, rememberBrowser: false);
return RedirectToLocal(returnUrl);
}
}
AddErrors(result);
}
ViewBag.ReturnUrl = returnUrl;
return View(model);
}
//
// POST: /Account/LogOff
[HttpPost]
[ValidateAntiForgeryToken]
public ActionResult LogOff()
{
AuthenticationManager.SignOut();
return RedirectToAction("Index", "Home");
}
//
// GET: /Account/ExternalLoginFailure
[AllowAnonymous]
public ActionResult ExternalLoginFailure()
{
return View();
}
protected override void Dispose(bool disposing)
{
if (disposing)
{
if (_userManager != null)
{
_userManager.Dispose();
_userManager = null;
}
if (_signInManager != null)
{
_signInManager.Dispose();
_signInManager = null;
}
}
base.Dispose(disposing);
}
#region Helpers
// Used for XSRF protection when adding external logins
private const string XsrfKey = "XsrfId";
private IAuthenticationManager AuthenticationManager
{
get
{
return HttpContext.GetOwinContext().Authentication;
}
}
private void AddErrors(IdentityResult result)
{
foreach (var error in result.Errors)
{
ModelState.AddModelError("", error);
}
}
private ActionResult RedirectToLocal(string returnUrl)
{
if (Url.IsLocalUrl(returnUrl))
{
return Redirect(returnUrl);
}
return RedirectToAction("Index", "Home");
}
internal class ChallengeResult : HttpUnauthorizedResult
{
public ChallengeResult(string provider, string redirectUri)
: this(provider, redirectUri, null)
{
}
public ChallengeResult(string provider, string redirectUri, string userId)
{
LoginProvider = provider;
RedirectUri = redirectUri;
UserId = userId;
}
public string LoginProvider { get; set; }
public string RedirectUri { get; set; }
public string UserId { get; set; }
public override void ExecuteResult(ControllerContext context)
{
var properties = new AuthenticationProperties { RedirectUri = RedirectUri };
if (UserId != null)
{
properties.Dictionary[XsrfKey] = UserId;
}
context.HttpContext.GetOwinContext().Authentication.Challenge(properties, LoginProvider);
}
}
#endregion
}
} | {'content_hash': 'e16a5921e5a03046d046aeb25f6ee32d', 'timestamp': '', 'source': 'github', 'line_count': 485, 'max_line_length': 173, 'avg_line_length': 35.74432989690722, 'alnum_prop': 0.5511652053530226, 'repo_name': 'ValerieRen/CSharpFinal', 'id': '837a333d51bd6927ab03bddada1f9b10ad8bafd3', 'size': '17338', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'Calculator/Model/Controllers/AccountController.cs', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'ASP', 'bytes': '96'}, {'name': 'C#', 'bytes': '113326'}, {'name': 'CSS', 'bytes': '513'}, {'name': 'HTML', 'bytes': '5127'}, {'name': 'JavaScript', 'bytes': '10714'}]} |
#ifndef itkGradientNDAnisotropicDiffusionFunction_h
#define itkGradientNDAnisotropicDiffusionFunction_h
#include "itkScalarAnisotropicDiffusionFunction.h"
#include "itkNeighborhoodAlgorithm.h"
#include "itkNeighborhoodInnerProduct.h"
#include "itkDerivativeOperator.h"
namespace itk
{
/** \class GradientNDAnisotropicDiffusionFunction
*
* This class implements an N-dimensional version of the classic Perona-Malik
* anisotropic diffusion equation for scalar-valued images. See
* itkAnisotropicDiffusionFunction for an overview of the anisotropic diffusion
* framework and equation.
*
* \par
* The conductance term for this implementation is chosen as a function of the
* gradient magnitude of the image at each point, reducing the strength of
* diffusion at edge pixels.
*
* \f[C(\mathbf{x}) = e^{-(\frac{\parallel \nabla U(\mathbf{x}) \parallel}{K})^2}\f].
*
* \par
* The numerical implementation of this equation is similar to that described
* in the Perona-Malik paper below, but uses a more robust technique
* for gradient magnitude estimation and has been generalized to N-dimensions.
*
* \par References
* Pietro Perona and Jalhandra Malik, ``Scale-space and edge detection using
* anisotropic diffusion,'' IEEE Transactions on Pattern Analysis Machine
* Intelligence, vol. 12, pp. 629-639, 1990.
*
* \sa AnisotropicDiffusionFunction
* \sa VectorAnisotropicDiffusionFunction
* \sa VectorGradientAnisotropicDiffusionFunction
* \sa CurvatureNDAnisotropicDiffusionFunction
* \ingroup FiniteDifferenceFunctions
* \ingroup ImageEnhancement
* \ingroup ITKAnisotropicSmoothing
*/
template< typename TImage >
class ITK_TEMPLATE_EXPORT GradientNDAnisotropicDiffusionFunction:
public ScalarAnisotropicDiffusionFunction< TImage >
{
public:
/** Standard class typedefs. */
typedef GradientNDAnisotropicDiffusionFunction Self;
typedef ScalarAnisotropicDiffusionFunction< TImage > Superclass;
typedef SmartPointer< Self > Pointer;
typedef SmartPointer< const Self > ConstPointer;
/** Method for creation through the object factory. */
itkNewMacro(Self);
/** Run-time type information (and related methods) */
itkTypeMacro(GradientNDAnisotropicDiffusionFunction,
ScalarAnisotropicDiffusionFunction);
/** Inherit some parameters from the superclass type. */
typedef typename Superclass::ImageType ImageType;
typedef typename Superclass::PixelType PixelType;
typedef typename Superclass::PixelRealType PixelRealType;
typedef typename Superclass::TimeStepType TimeStepType;
typedef typename Superclass::RadiusType RadiusType;
typedef typename Superclass::NeighborhoodType NeighborhoodType;
typedef typename Superclass::FloatOffsetType FloatOffsetType;
typedef SizeValueType NeighborhoodSizeValueType;
/** Inherit some parameters from the superclass type. */
itkStaticConstMacro(ImageDimension, unsigned int, Superclass::ImageDimension);
/** Compute the equation value. */
virtual PixelType ComputeUpdate(const NeighborhoodType & neighborhood,
void *globalData,
const FloatOffsetType & offset = FloatOffsetType(0.0)
) ITK_OVERRIDE;
/** This method is called prior to each iteration of the solver. */
virtual void InitializeIteration() ITK_OVERRIDE
{
m_K = static_cast< PixelType >( this->GetAverageGradientMagnitudeSquared()
* this->GetConductanceParameter() * this->GetConductanceParameter() * -2.0f );
}
protected:
GradientNDAnisotropicDiffusionFunction();
~GradientNDAnisotropicDiffusionFunction() {}
/** Inner product function. */
NeighborhoodInnerProduct< ImageType > m_InnerProduct;
/** Slices for the ND neighborhood. */
std::slice x_slice[ImageDimension];
std::slice xa_slice[ImageDimension][ImageDimension];
std::slice xd_slice[ImageDimension][ImageDimension];
/** Derivative operator. */
DerivativeOperator< PixelType, itkGetStaticConstMacro(ImageDimension) > dx_op;
/** Modified global average gradient magnitude term. */
PixelType m_K;
NeighborhoodSizeValueType m_Center;
NeighborhoodSizeValueType m_Stride[ImageDimension];
static double m_MIN_NORM;
private:
ITK_DISALLOW_COPY_AND_ASSIGN(GradientNDAnisotropicDiffusionFunction);
};
} // end namespace itk
#ifndef ITK_MANUAL_INSTANTIATION
#include "itkGradientNDAnisotropicDiffusionFunction.hxx"
#endif
#endif
| {'content_hash': 'c422a09fba867d425cea6e4503e9171f', 'timestamp': '', 'source': 'github', 'line_count': 121, 'max_line_length': 114, 'avg_line_length': 38.429752066115704, 'alnum_prop': 0.7264516129032258, 'repo_name': 'RayRuizhiLiao/ITK_4D', 'id': '1e1e4f28a9fc8ef0fd197d3b3cb1d663722cd5f5', 'size': '5441', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'Modules/Filtering/AnisotropicSmoothing/include/itkGradientNDAnisotropicDiffusionFunction.h', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'C', 'bytes': '572693'}, {'name': 'C++', 'bytes': '36720665'}, {'name': 'CMake', 'bytes': '1448020'}, {'name': 'CSS', 'bytes': '18346'}, {'name': 'Java', 'bytes': '29480'}, {'name': 'Objective-C++', 'bytes': '6753'}, {'name': 'Perl', 'bytes': '6113'}, {'name': 'Python', 'bytes': '385395'}, {'name': 'Ruby', 'bytes': '309'}, {'name': 'Shell', 'bytes': '92050'}, {'name': 'Tcl', 'bytes': '75202'}, {'name': 'XSLT', 'bytes': '8874'}]} |
<?php
namespace Vivo\Form\Element;
use Zend\Form\ElementPrepareAwareInterface;
use Zend\Form\FormInterface;
use Zend\Form\Element\Button as ZendButton;
class Button extends ZendButton implements ElementPrepareAwareInterface
{
/**
* Prepare the form element (mostly used for rendering purposes)
* @param FormInterface $form
* @return mixed
*/
public function prepareElement(FormInterface $form)
{
//Add 'id' attribute
if (!$this->getAttribute('id')) {
$id = str_replace(']', '', $this->getName());
$id = str_replace('[', '-', $id);
$this->setAttribute('id', $id);
}
}
}
| {'content_hash': 'eef61c748c4c644d6a3df1820b53a93b', 'timestamp': '', 'source': 'github', 'line_count': 24, 'max_line_length': 71, 'avg_line_length': 27.791666666666668, 'alnum_prop': 0.6176911544227887, 'repo_name': 'miroslavhajek/vivoportal', 'id': 'aa4f33e31c3bb94f66e0c720f470aa6192071891', 'size': '667', 'binary': False, 'copies': '1', 'ref': 'refs/heads/develop', 'path': 'src/Vivo/Form/Element/Button.php', 'mode': '33188', 'license': 'bsd-3-clause', 'language': [{'name': 'CSS', 'bytes': '435160'}, {'name': 'JavaScript', 'bytes': '399518'}, {'name': 'PHP', 'bytes': '1732609'}]} |
var limit = 10;
var items = [{v: 0, w: 1}, {v: 2, w: 2}, {v: 3, w: 3}, {v: 4, w: 4}, {v: 5, w: 5}];
var collection = function(items, weight, value) {
(items) ? this.items = items.slice() : this.items = [];
this.weight = weight || 0;
this.value = value || 0;
};
function permute(c, index) {
if (c.weight + items[index].w > limit) return c;
c.items.push(index); c.weight += items[index].w; c.value += items[index].v;
var coll, coll_largest;
for (var i = 0; i < items.length; i++) {
coll = permute(new collection(c.items, c.weight, c.value), i);
if (typeof coll_largest == 'undefined' || coll.value > coll_largest.value) {
coll_largest = coll;
}
}
return coll_largest;
}
console.log(permute(new collection(), 0));
| {'content_hash': '48cce4f15bbfde8975c4389575b67cbf', 'timestamp': '', 'source': 'github', 'line_count': 23, 'max_line_length': 84, 'avg_line_length': 34.17391304347826, 'alnum_prop': 0.5623409669211196, 'repo_name': 'MrHamdulay/daily-problems', 'id': '48b67558546f677233000fe1e10f504831b51fe5', 'size': '786', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'jarred/problem-5-max-weight-value.js', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'JavaScript', 'bytes': '5949'}, {'name': 'Python', 'bytes': '3034'}]} |
package apple.scenekit;
import apple.NSObject;
import apple.foundation.NSArray;
import apple.foundation.NSCoder;
import apple.foundation.NSMethodSignature;
import apple.foundation.NSSet;
import apple.scenekit.struct.SCNVector3;
import org.moe.natj.c.ann.FunctionPtr;
import org.moe.natj.general.NatJ;
import org.moe.natj.general.Pointer;
import org.moe.natj.general.ann.ByValue;
import org.moe.natj.general.ann.Generated;
import org.moe.natj.general.ann.Library;
import org.moe.natj.general.ann.Mapped;
import org.moe.natj.general.ann.NFloat;
import org.moe.natj.general.ann.NInt;
import org.moe.natj.general.ann.NUInt;
import org.moe.natj.general.ann.Owned;
import org.moe.natj.general.ann.Runtime;
import org.moe.natj.general.ptr.VoidPtr;
import org.moe.natj.objc.Class;
import org.moe.natj.objc.ObjCRuntime;
import org.moe.natj.objc.SEL;
import org.moe.natj.objc.ann.ObjCClassBinding;
import org.moe.natj.objc.ann.ProtocolClassMethod;
import org.moe.natj.objc.ann.Selector;
import org.moe.natj.objc.map.ObjCObjectMapper;
/**
* SCNIKConstraint
* <p>
* A SCNIKConstraint applies an inverse kinematics constraint
*/
@Generated
@Library("SceneKit")
@Runtime(ObjCRuntime.class)
@ObjCClassBinding
public class SCNIKConstraint extends SCNConstraint {
static {
NatJ.register();
}
@Generated
protected SCNIKConstraint(Pointer peer) {
super(peer);
}
@Generated
@Selector("accessInstanceVariablesDirectly")
public static native boolean accessInstanceVariablesDirectly();
@Generated
@Owned
@Selector("alloc")
public static native SCNIKConstraint alloc();
@Owned
@Generated
@Selector("allocWithZone:")
public static native SCNIKConstraint allocWithZone(VoidPtr zone);
@Generated
@Selector("automaticallyNotifiesObserversForKey:")
public static native boolean automaticallyNotifiesObserversForKey(String key);
@Generated
@Selector("cancelPreviousPerformRequestsWithTarget:")
public static native void cancelPreviousPerformRequestsWithTarget(@Mapped(ObjCObjectMapper.class) Object aTarget);
@Generated
@Selector("cancelPreviousPerformRequestsWithTarget:selector:object:")
public static native void cancelPreviousPerformRequestsWithTargetSelectorObject(
@Mapped(ObjCObjectMapper.class) Object aTarget, SEL aSelector,
@Mapped(ObjCObjectMapper.class) Object anArgument);
@Generated
@Selector("classFallbacksForKeyedArchiver")
public static native NSArray<String> classFallbacksForKeyedArchiver();
@Generated
@Selector("classForKeyedUnarchiver")
public static native Class classForKeyedUnarchiver();
@Generated
@Selector("debugDescription")
public static native String debugDescription_static();
@Generated
@Selector("description")
public static native String description_static();
@Generated
@Selector("hash")
@NUInt
public static native long hash_static();
@Generated
@Selector("instanceMethodForSelector:")
@FunctionPtr(name = "call_instanceMethodForSelector_ret")
public static native NSObject.Function_instanceMethodForSelector_ret instanceMethodForSelector(SEL aSelector);
@Generated
@Selector("instanceMethodSignatureForSelector:")
public static native NSMethodSignature instanceMethodSignatureForSelector(SEL aSelector);
@Generated
@Selector("instancesRespondToSelector:")
public static native boolean instancesRespondToSelector(SEL aSelector);
/**
* inverseKinematicsConstraintWithChainRootNode:
* <p>
* Creates and returns a SCNIKConstraint object with the specified parameter.
* <p>
* "chainRootNode" must be an ancestor of the node on which the constraint is applied.
*
* @param chainRootNode The root node of the kinematic chain.
*/
@Generated
@Selector("inverseKinematicsConstraintWithChainRootNode:")
public static native SCNIKConstraint inverseKinematicsConstraintWithChainRootNode(SCNNode chainRootNode);
@Generated
@Selector("isSubclassOfClass:")
public static native boolean isSubclassOfClass(Class aClass);
@Generated
@Selector("keyPathsForValuesAffectingValueForKey:")
public static native NSSet<String> keyPathsForValuesAffectingValueForKey(String key);
@Generated
@Owned
@Selector("new")
public static native SCNIKConstraint new_objc();
@Generated
@Selector("resolveClassMethod:")
public static native boolean resolveClassMethod(SEL sel);
@Generated
@Selector("resolveInstanceMethod:")
public static native boolean resolveInstanceMethod(SEL sel);
@Generated
@Selector("setVersion:")
public static native void setVersion_static(@NInt long aVersion);
@Generated
@Selector("superclass")
public static native Class superclass_static();
@Generated
@Selector("supportsSecureCoding")
public static native boolean supportsSecureCoding();
@Generated
@Selector("version")
@NInt
public static native long version_static();
/**
* [@property] chainRootNode
* <p>
* Specifies the root node of the kinematic chain.
*/
@Generated
@Selector("chainRootNode")
public native SCNNode chainRootNode();
@Generated
@Selector("init")
public native SCNIKConstraint init();
/**
* initWithChainRootNode:
* <p>
* Creates and returns a SCNIKConstraint object with the specified parameter.
* <p>
* "chainRootNode" must be an ancestor of the node on which the constraint is applied.
*
* @param chainRootNode The root node of the kinematic chain.
*/
@Generated
@Selector("initWithChainRootNode:")
public native SCNIKConstraint initWithChainRootNode(SCNNode chainRootNode);
@Generated
@Selector("initWithCoder:")
public native SCNIKConstraint initWithCoder(NSCoder coder);
@Generated
@Selector("maxAllowedRotationAngleForJoint:")
@NFloat
public native double maxAllowedRotationAngleForJoint(SCNNode node);
/**
* setMaxAllowedRotationAngle:forJoint:
* <p>
* Specifies the maximum rotation allowed (in degrees) for the specified joint from its initial orientation.
* Defaults to 180.
*/
@Generated
@Selector("setMaxAllowedRotationAngle:forJoint:")
public native void setMaxAllowedRotationAngleForJoint(@NFloat double angle, SCNNode node);
/**
* [@property] target
* <p>
* Specifies the target position (in world space coordinates) of the end joint (i.e the node that owns the IK
* constraint). Defaults to (0,0,0). Animatable.
*/
@Generated
@Selector("setTargetPosition:")
public native void setTargetPosition(@ByValue SCNVector3 value);
@Generated
@ProtocolClassMethod("supportsSecureCoding")
public boolean _supportsSecureCoding() {
return supportsSecureCoding();
}
/**
* [@property] target
* <p>
* Specifies the target position (in world space coordinates) of the end joint (i.e the node that owns the IK
* constraint). Defaults to (0,0,0). Animatable.
*/
@Generated
@Selector("targetPosition")
@ByValue
public native SCNVector3 targetPosition();
}
| {'content_hash': '6f0eb412fe05c33f4fd7379613a68cd9', 'timestamp': '', 'source': 'github', 'line_count': 235, 'max_line_length': 118, 'avg_line_length': 30.91063829787234, 'alnum_prop': 0.7293502202643172, 'repo_name': 'multi-os-engine/moe-core', 'id': '4bca7e416a2661c84538f13a4de4e75478a75921', 'size': '7832', 'binary': False, 'copies': '1', 'ref': 'refs/heads/moe-master', 'path': 'moe.apple/moe.platform.ios/src/main/java/apple/scenekit/SCNIKConstraint.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'C', 'bytes': '892337'}, {'name': 'C++', 'bytes': '155476'}, {'name': 'HTML', 'bytes': '42061'}, {'name': 'Java', 'bytes': '46670080'}, {'name': 'Objective-C', 'bytes': '94699'}, {'name': 'Objective-C++', 'bytes': '9128'}, {'name': 'Perl', 'bytes': '35206'}, {'name': 'Python', 'bytes': '15418'}, {'name': 'Shell', 'bytes': '10932'}]} |
<?php
/**
* Generated by Protobuf protoc plugin.
*
* File descriptor : POGOProtos/Settings/GpsSettings.proto
*/
namespace POGOProtos\Settings;
/**
* Protobuf message : POGOProtos.Settings.GpsSettings
*/
class GpsSettings extends \Protobuf\AbstractMessage
{
/**
* @var \Protobuf\UnknownFieldSet
*/
protected $unknownFieldSet = null;
/**
* @var \Protobuf\Extension\ExtensionFieldMap
*/
protected $extensions = null;
/**
* driving_warning_speed_meters_per_second optional float = 1
*
* @var float
*/
protected $driving_warning_speed_meters_per_second = null;
/**
* driving_warning_cooldown_minutes optional float = 2
*
* @var float
*/
protected $driving_warning_cooldown_minutes = null;
/**
* driving_speed_sample_interval_seconds optional float = 3
*
* @var float
*/
protected $driving_speed_sample_interval_seconds = null;
/**
* driving_speed_sample_count optional int32 = 4
*
* @var int
*/
protected $driving_speed_sample_count = null;
/**
* Check if 'driving_warning_speed_meters_per_second' has a value
*
* @return bool
*/
public function hasDrivingWarningSpeedMetersPerSecond()
{
return $this->driving_warning_speed_meters_per_second !== null;
}
/**
* Get 'driving_warning_speed_meters_per_second' value
*
* @return float
*/
public function getDrivingWarningSpeedMetersPerSecond()
{
return $this->driving_warning_speed_meters_per_second;
}
/**
* Set 'driving_warning_speed_meters_per_second' value
*
* @param float $value
*/
public function setDrivingWarningSpeedMetersPerSecond($value = null)
{
$this->driving_warning_speed_meters_per_second = $value;
}
/**
* Check if 'driving_warning_cooldown_minutes' has a value
*
* @return bool
*/
public function hasDrivingWarningCooldownMinutes()
{
return $this->driving_warning_cooldown_minutes !== null;
}
/**
* Get 'driving_warning_cooldown_minutes' value
*
* @return float
*/
public function getDrivingWarningCooldownMinutes()
{
return $this->driving_warning_cooldown_minutes;
}
/**
* Set 'driving_warning_cooldown_minutes' value
*
* @param float $value
*/
public function setDrivingWarningCooldownMinutes($value = null)
{
$this->driving_warning_cooldown_minutes = $value;
}
/**
* Check if 'driving_speed_sample_interval_seconds' has a value
*
* @return bool
*/
public function hasDrivingSpeedSampleIntervalSeconds()
{
return $this->driving_speed_sample_interval_seconds !== null;
}
/**
* Get 'driving_speed_sample_interval_seconds' value
*
* @return float
*/
public function getDrivingSpeedSampleIntervalSeconds()
{
return $this->driving_speed_sample_interval_seconds;
}
/**
* Set 'driving_speed_sample_interval_seconds' value
*
* @param float $value
*/
public function setDrivingSpeedSampleIntervalSeconds($value = null)
{
$this->driving_speed_sample_interval_seconds = $value;
}
/**
* Check if 'driving_speed_sample_count' has a value
*
* @return bool
*/
public function hasDrivingSpeedSampleCount()
{
return $this->driving_speed_sample_count !== null;
}
/**
* Get 'driving_speed_sample_count' value
*
* @return int
*/
public function getDrivingSpeedSampleCount()
{
return $this->driving_speed_sample_count;
}
/**
* Set 'driving_speed_sample_count' value
*
* @param int $value
*/
public function setDrivingSpeedSampleCount($value = null)
{
$this->driving_speed_sample_count = $value;
}
/**
* {@inheritdoc}
*/
public function extensions()
{
if ( $this->extensions !== null) {
return $this->extensions;
}
return $this->extensions = new \Protobuf\Extension\ExtensionFieldMap(__CLASS__);
}
/**
* {@inheritdoc}
*/
public function unknownFieldSet()
{
return $this->unknownFieldSet;
}
/**
* {@inheritdoc}
*/
public static function fromStream($stream, \Protobuf\Configuration $configuration = null)
{
return new self($stream, $configuration);
}
/**
* {@inheritdoc}
*/
public static function fromArray(array $values)
{
$message = new self();
$values = array_merge([
'driving_warning_speed_meters_per_second' => null,
'driving_warning_cooldown_minutes' => null,
'driving_speed_sample_interval_seconds' => null,
'driving_speed_sample_count' => null
], $values);
$message->setDrivingWarningSpeedMetersPerSecond($values['driving_warning_speed_meters_per_second']);
$message->setDrivingWarningCooldownMinutes($values['driving_warning_cooldown_minutes']);
$message->setDrivingSpeedSampleIntervalSeconds($values['driving_speed_sample_interval_seconds']);
$message->setDrivingSpeedSampleCount($values['driving_speed_sample_count']);
return $message;
}
/**
* {@inheritdoc}
*/
public static function descriptor()
{
return \google\protobuf\DescriptorProto::fromArray([
'name' => 'GpsSettings',
'field' => [
\google\protobuf\FieldDescriptorProto::fromArray([
'number' => 1,
'name' => 'driving_warning_speed_meters_per_second',
'type' => \google\protobuf\FieldDescriptorProto\Type::TYPE_FLOAT(),
'label' => \google\protobuf\FieldDescriptorProto\Label::LABEL_OPTIONAL()
]),
\google\protobuf\FieldDescriptorProto::fromArray([
'number' => 2,
'name' => 'driving_warning_cooldown_minutes',
'type' => \google\protobuf\FieldDescriptorProto\Type::TYPE_FLOAT(),
'label' => \google\protobuf\FieldDescriptorProto\Label::LABEL_OPTIONAL()
]),
\google\protobuf\FieldDescriptorProto::fromArray([
'number' => 3,
'name' => 'driving_speed_sample_interval_seconds',
'type' => \google\protobuf\FieldDescriptorProto\Type::TYPE_FLOAT(),
'label' => \google\protobuf\FieldDescriptorProto\Label::LABEL_OPTIONAL()
]),
\google\protobuf\FieldDescriptorProto::fromArray([
'number' => 4,
'name' => 'driving_speed_sample_count',
'type' => \google\protobuf\FieldDescriptorProto\Type::TYPE_INT32(),
'label' => \google\protobuf\FieldDescriptorProto\Label::LABEL_OPTIONAL()
]),
],
]);
}
/**
* {@inheritdoc}
*/
public function toStream(\Protobuf\Configuration $configuration = null)
{
$config = $configuration ?: \Protobuf\Configuration::getInstance();
$context = $config->createWriteContext();
$stream = $context->getStream();
$this->writeTo($context);
$stream->seek(0);
return $stream;
}
/**
* {@inheritdoc}
*/
public function writeTo(\Protobuf\WriteContext $context)
{
$stream = $context->getStream();
$writer = $context->getWriter();
$sizeContext = $context->getComputeSizeContext();
if ($this->driving_warning_speed_meters_per_second !== null) {
$writer->writeVarint($stream, 13);
$writer->writeFloat($stream, $this->driving_warning_speed_meters_per_second);
}
if ($this->driving_warning_cooldown_minutes !== null) {
$writer->writeVarint($stream, 21);
$writer->writeFloat($stream, $this->driving_warning_cooldown_minutes);
}
if ($this->driving_speed_sample_interval_seconds !== null) {
$writer->writeVarint($stream, 29);
$writer->writeFloat($stream, $this->driving_speed_sample_interval_seconds);
}
if ($this->driving_speed_sample_count !== null) {
$writer->writeVarint($stream, 32);
$writer->writeVarint($stream, $this->driving_speed_sample_count);
}
if ($this->extensions !== null) {
$this->extensions->writeTo($context);
}
return $stream;
}
/**
* {@inheritdoc}
*/
public function readFrom(\Protobuf\ReadContext $context)
{
$reader = $context->getReader();
$length = $context->getLength();
$stream = $context->getStream();
$limit = ($length !== null)
? ($stream->tell() + $length)
: null;
while ($limit === null || $stream->tell() < $limit) {
if ($stream->eof()) {
break;
}
$key = $reader->readVarint($stream);
$wire = \Protobuf\WireFormat::getTagWireType($key);
$tag = \Protobuf\WireFormat::getTagFieldNumber($key);
if ($stream->eof()) {
break;
}
if ($tag === 1) {
\Protobuf\WireFormat::assertWireType($wire, 2);
$this->driving_warning_speed_meters_per_second = $reader->readFloat($stream);
continue;
}
if ($tag === 2) {
\Protobuf\WireFormat::assertWireType($wire, 2);
$this->driving_warning_cooldown_minutes = $reader->readFloat($stream);
continue;
}
if ($tag === 3) {
\Protobuf\WireFormat::assertWireType($wire, 2);
$this->driving_speed_sample_interval_seconds = $reader->readFloat($stream);
continue;
}
if ($tag === 4) {
\Protobuf\WireFormat::assertWireType($wire, 5);
$this->driving_speed_sample_count = $reader->readVarint($stream);
continue;
}
$extensions = $context->getExtensionRegistry();
$extension = $extensions ? $extensions->findByNumber(__CLASS__, $tag) : null;
if ($extension !== null) {
$this->extensions()->add($extension, $extension->readFrom($context, $wire));
continue;
}
if ($this->unknownFieldSet === null) {
$this->unknownFieldSet = new \Protobuf\UnknownFieldSet();
}
$data = $reader->readUnknown($stream, $wire);
$unknown = new \Protobuf\Unknown($tag, $wire, $data);
$this->unknownFieldSet->add($unknown);
}
}
/**
* {@inheritdoc}
*/
public function serializedSize(\Protobuf\ComputeSizeContext $context)
{
$calculator = $context->getSizeCalculator();
$size = 0;
if ($this->driving_warning_speed_meters_per_second !== null) {
$size += 1;
$size += 4;
}
if ($this->driving_warning_cooldown_minutes !== null) {
$size += 1;
$size += 4;
}
if ($this->driving_speed_sample_interval_seconds !== null) {
$size += 1;
$size += 4;
}
if ($this->driving_speed_sample_count !== null) {
$size += 1;
$size += $calculator->computeVarintSize($this->driving_speed_sample_count);
}
if ($this->extensions !== null) {
$size += $this->extensions->serializedSize($context);
}
return $size;
}
/**
* {@inheritdoc}
*/
public function clear()
{
$this->driving_warning_speed_meters_per_second = null;
$this->driving_warning_cooldown_minutes = null;
$this->driving_speed_sample_interval_seconds = null;
$this->driving_speed_sample_count = null;
}
/**
* {@inheritdoc}
*/
public function merge(\Protobuf\Message $message)
{
if ( ! $message instanceof \POGOProtos\Settings\GpsSettings) {
throw new \InvalidArgumentException(sprintf('Argument 1 passed to %s must be a %s, %s given', __METHOD__, __CLASS__, get_class($message)));
}
$this->driving_warning_speed_meters_per_second = ($message->driving_warning_speed_meters_per_second !== null) ? $message->driving_warning_speed_meters_per_second : $this->driving_warning_speed_meters_per_second;
$this->driving_warning_cooldown_minutes = ($message->driving_warning_cooldown_minutes !== null) ? $message->driving_warning_cooldown_minutes : $this->driving_warning_cooldown_minutes;
$this->driving_speed_sample_interval_seconds = ($message->driving_speed_sample_interval_seconds !== null) ? $message->driving_speed_sample_interval_seconds : $this->driving_speed_sample_interval_seconds;
$this->driving_speed_sample_count = ($message->driving_speed_sample_count !== null) ? $message->driving_speed_sample_count : $this->driving_speed_sample_count;
}
}
| {'content_hash': 'd2e3db80d916cca4a7222502e2e7be87', 'timestamp': '', 'source': 'github', 'line_count': 454, 'max_line_length': 219, 'avg_line_length': 29.36123348017621, 'alnum_prop': 0.5619654913728432, 'repo_name': 'jaspervdm/pogoprotos-php', 'id': '087d561e3c1ed1db2f66864cd841ea2fff6e2122', 'size': '13330', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/POGOProtos/Settings/GpsSettings.php', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'PHP', 'bytes': '4873465'}]} |
var http = require('http');
var express = require('express');
var ecstatic = require('ecstatic');
var app = express();
app.use(ecstatic({
root: __dirname,
gzip: true
}));
http.createServer(app).listen(3000);
console.log('Listening on port 3000'); | {'content_hash': '5cd8b932ed90e61f9730084a0c3aa577', 'timestamp': '', 'source': 'github', 'line_count': 10, 'max_line_length': 38, 'avg_line_length': 25.0, 'alnum_prop': 0.688, 'repo_name': 'darrencruse/sugarlisp-plus', 'id': '6623f84d05621cd0ac83ab0de26e4e4c84f2ac2d', 'size': '283', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'examples/ecstatic.js', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'JavaScript', 'bytes': '26064'}, {'name': 'Shell', 'bytes': '1775'}]} |
elisp
=====
This is my config environment of Emacs.
| {'content_hash': 'e2fa11bf0276b77dc2e233e397e703af', 'timestamp': '', 'source': 'github', 'line_count': 4, 'max_line_length': 39, 'avg_line_length': 13.25, 'alnum_prop': 0.6981132075471698, 'repo_name': 'lyanchih/elisp', 'id': '17c6b7f454bc467db0664c104628ec0cf32660cc', 'size': '53', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'README.md', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Awk', 'bytes': '500'}, {'name': 'C', 'bytes': '13276'}, {'name': 'C++', 'bytes': '43359'}, {'name': 'Common Lisp', 'bytes': '1172924'}, {'name': 'Emacs Lisp', 'bytes': '5743630'}, {'name': 'Fortran', 'bytes': '2529'}, {'name': 'Java', 'bytes': '1897'}, {'name': 'Makefile', 'bytes': '62477'}, {'name': 'Ruby', 'bytes': '7652'}, {'name': 'SRecode Template', 'bytes': '55991'}, {'name': 'Scheme', 'bytes': '131159'}, {'name': 'Shell', 'bytes': '5384'}, {'name': 'TeX', 'bytes': '3118'}]} |
using System;
using System.IO;
using Udpc.Share.Internal;
namespace Udpc.Share.DataLog
{
public class FileDataItem : DataLogItem
{
public byte[] Content { get; private set; }
public long Offset { get; private set; }
public FileDataItem(Guid itemid, byte[] content, long offset) : base(itemid)
{
Content = content;
Offset = offset;
}
public override string ToString()
{
return $"FileData {Offset} {Content.Length}";
}
public override void Write(Stream stream)
{
stream.WriteLong(Offset);
stream.WriteLong(Content.Length);
stream.Write(Content);
}
public override void Read(Stream stream)
{
Offset = stream.ReadLong();
Content = new byte[stream.ReadLong()];
stream.Read(Content, 0, Content.Length);
}
}
} | {'content_hash': '6b547735c79bb56b1800a07fbd4e8f78', 'timestamp': '', 'source': 'github', 'line_count': 37, 'max_line_length': 84, 'avg_line_length': 25.405405405405407, 'alnum_prop': 0.5585106382978723, 'repo_name': 'rolfrm/UDP-Client', 'id': '063c3b0bc0bf0b8c9716976e6d26151b10583e0b', 'size': '942', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'Udpc.Share/Udpc.Share/DataLog/FileDataItem.cs', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'C', 'bytes': '230004'}, {'name': 'C#', 'bytes': '112934'}, {'name': 'C++', 'bytes': '475'}, {'name': 'CSS', 'bytes': '1480'}, {'name': 'HTML', 'bytes': '4124'}, {'name': 'JavaScript', 'bytes': '142'}, {'name': 'Makefile', 'bytes': '2274'}, {'name': 'Shell', 'bytes': '120'}]} |
namespace lia {
template<class Cont, typename T, class BinaryFunc>
inline T foldl(Cont&& cont, BinaryFunc&& func, T starting) {
return std::accumulate(std::begin(std::forward<Cont>(cont)),
std::end(std::forward<Cont>(cont)),
starting,
func);
}
template<class Cont, typename T, class BinaryFunc>
inline T foldr(Cont&& cont, BinaryFunc&& func, T starting) {
auto first = rbegin(std::forward<Cont>(cont));
auto last = rend(std::forward<Cont>(cont));
for(; first != last; ++first)
starting = func(*first, starting);
return starting;
}
template<class Cont, class BinaryFunc>
inline auto foldl1(Cont&& cont, BinaryFunc&& func) -> decltype(func(cont.back(), cont.back())) {
auto first = std::begin(cont);
auto last = std::end(cont);
decltype(func(cont.back(), cont.back())) init = *first++;
for(; first != last; ++first)
init = func(init, *first);
return init;
}
template<class Cont, class BinaryFunc>
inline auto foldr1(Cont&& cont, BinaryFunc&& func) -> decltype(func(cont.back(), cont.back())) {
auto first = rbegin(cont);
auto last = rend(cont);
decltype(func(cont.back(), cont.back())) init = *first++;
for(; first != last; ++first)
init = func(*first, init);
return init;
}
// Special folds
template<class Cont>
inline ValueType<Cont> sum(Cont&& cont) {
using T = ValueType<Cont>;
return foldl1(std::forward<Cont>(cont), [](T x, T y) { return x + y; });
}
template<class Cont>
inline ValueType<Cont> product(Cont&& cont) {
using T = ValueType<Cont>;
return foldl1(std::forward<Cont>(cont), [](T x, T y) { return x * y; });
}
template<class Cont, class Predicate = less>
inline ValueType<Cont> minimum(Cont&& cont, Predicate&& pred = Predicate()) {
return *(std::min_element(std::begin(std::forward<Cont>(cont)),
std::end(std::forward<Cont>(cont)),
std::forward<Predicate>(pred)));
}
template<class Cont, class Predicate = less>
inline ValueType<Cont> maximum(Cont&& cont, Predicate&& pred = Predicate()) {
return *(std::max_element(std::begin(std::forward<Cont>(cont)),
std::end(std::forward<Cont>(cont)),
std::forward<Predicate>(pred)));
}
template<class Cont>
inline bool all(Cont&& cont) {
static_assert(std::is_convertible<ValueType<Cont>, bool>(), "\"all\" special fold requires boolean conversion");
for(auto&& boolean : cont) {
if(!boolean)
return false;
}
return true;
}
template<class Cont, class Predicate>
inline bool all(Cont&& cont, Predicate&& pred) {
return std::all_of(std::begin(std::forward<Cont>(cont)),
std::end(std::forward<Cont>(cont)),
std::forward<Predicate>(pred));
}
template<class Cont>
inline bool any(Cont&& cont) {
static_assert(std::is_convertible<ValueType<Cont>, bool>(), "\"any\" special fold requires boolean conversion");
for(auto&& boolean : cont) {
if(boolean)
return true;
}
return false;
}
template<class Cont, class Predicate>
inline bool any(Cont&& cont, Predicate&& pred) {
return std::any_of(std::begin(std::forward<Cont>(cont)),
std::end(std::forward<Cont>(cont)),
std::forward<Predicate>(pred));
}
template<class Cont, DisableIf<has_append<ValueType<Cont>>>...>
inline auto concat(Cont&& cont) -> Rebind<Unqualified<Cont>, NestedValueType<Cont>> {
Rebind<Unqualified<Cont>, NestedValueType<Cont>> result;
for(auto&& internal : cont) {
result.insert(std::end(result), std::begin(internal), std::end(internal));
}
return result;
}
template<class Cont, EnableIf<has_append<ValueType<Cont>>>...>
inline auto concat(Cont&& cont) -> ValueType<Cont> {
ValueType<Cont> result;
for(auto&& str : cont)
result.append(str);
return result;
}
template<class Cont, class T = ValueType<Cont>>
T intercalate(Cont&& cont, T&& sublist) {
return concat(intersperse(std::forward<Cont>(cont), std::forward<T>(sublist)));
}
template<class Cont, class Callable, EnableIf<is_nested_container<Unqualified<Cont>>>...>
inline auto concat_map(Cont&& cont, Callable&& callable) -> decltype(concat(map(std::forward<Cont>(cont), std::forward<Callable>(callable)))) {
return concat(map(std::forward<Cont>(cont), std::forward<Callable>(callable)));
}
template<class Cont, class BinaryFunc, typename T>
inline auto scanl(Cont&& cont, BinaryFunc&& f, T acc) -> Rebind<Unqualified<Cont>, decltype(f(acc, cont.back()))> {
Rebind<Unqualified<Cont>, decltype(f(acc, cont.back()))> result;
auto first = std::begin(cont);
auto last = std::end(cont);
result.push_back(acc);
for( ; first != last; ++first) {
acc = f(acc, *first);
result.push_back(acc);
}
return result;
}
template<class Cont, class BinaryFunc>
inline auto scanl1(Cont&& cont, BinaryFunc&& f) -> Rebind<Unqualified<Cont>, decltype(f(cont.back(), cont.back()))> {
Rebind<Unqualified<Cont>, decltype(f(cont.back(), cont.back()))> result;
auto first = std::begin(cont);
auto last = std::end(cont);
decltype(f(cont.back(), cont.back())) acc = *first++;
result.push_back(acc);
for( ; first != last; ++first) {
acc = f(acc, *first);
result.push_back(acc);
}
return result;
}
} // lia
#endif // LIA_FOLDS_LIST_COMP_HPP | {'content_hash': 'e3d47b3612006e5830c905fe2cca773d', 'timestamp': '', 'source': 'github', 'line_count': 155, 'max_line_length': 143, 'avg_line_length': 35.619354838709675, 'alnum_prop': 0.6189096178228581, 'repo_name': 'Rapptz/Lia', 'id': '43a176758387692f110002baf25213c8a02e48f6', 'size': '5613', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'Lia/list/folds.hpp', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'C++', 'bytes': '47126'}]} |
<!doctype html>
<html ng-app="app">
<head>
<meta http-equiv="content-type" content="text/html; charset=utf-8">
<title>Materials</title>
<link rel="stylesheet" href="./css/bootstrap.css" media="screen">
</head>
<body>
<div class="container" ng-controller="MaterialsController">
<nav class="navbar navbar-default">
<div class="container-fluid">
<div class="navbar-header">
<button class="navbar-toggle collapsed" type="button"
data-toggle="collapse" data-target="#bs-example-navbar-collapse-1">
<span class="sr-only">Toggle navigation</span> <span
class="icon-bar"></span> <span class="icon-bar"></span> <span
class="icon-bar"></span>
</button>
<a class="navbar-brand" href="/">Storage Меринджей</a>
</div>
<div class="collapse navbar-collapse"
id="bs-example-navbar-collapse-1">
<ul class="nav navbar-nav">
<li class="active"><a href="materials">Materials</a></li>
<li class="active"><a href="deliveries">Deliveries</a></li>
<li class="active"><a href="vendors">Vendors <span
class="sr-only">(current)</span></a></li>
</ul>
<form class="navbar-form navbar-left" role="search">
<div class="form-group">
<input class="form-control" type="text" placeholder="Search">
</div>
<button class="btn btn-default" type="submit">Submit</button>
</form>
<ul class="nav navbar-nav navbar-right">
<li><a href="#">Link</a></li>
</ul>
</div>
</div>
</nav>
<table class="table table-striped table-hover ">
<thead>
<tr>
<th>#</th>
<th>Material</th>
<th>Quantity</th>
<th>Use</th>
</tr>
</thead>
<tbody>
<tr ng-repeat="material in materials">
<td>{{ $index + 1}}</td>
<td>{{ material.name }}</td>
<td>{{ material.quantity }}</td>
<td><input type="text" id="use{{ material.name }}" />
<button class="btn btn-default" type="submit" ng-click="useMaterial(material.name)">Use</button></td>
</tr>
</tbody>
</table>
<div>
<div class="well bs-component">
<form class="form-horizontal">
<fieldset>
<legend>Add new material</legend>
<div class="form-group">
<label for="inputName" class="col-lg-2 control-label">Name</label>
<div class="col-lg-10">
<input type="text" class="form-control" id="inputName"
ng-model="materialName" />
</div>
</div>
<div class="form-group">
<div class="col-lg-10 col-lg-offset-2">
<button type="submit" class="btn btn-primary"
ng-click="submit()">Submit</button>
</div>
</div>
</fieldset>
</form>
<div id="source-button" class="btn btn-primary btn-xs"
style="display: none;">< ></div>
</div>
</div>
<script src="./js/jquery-1.10.2.min.js"></script>
<script src="./js/bootstrap.min.js"></script>
<script
src="https://cdnjs.cloudflare.com/ajax/libs/angular.js/1.0.8/angular.js"></script>
<script src="./js/app.js"></script>
<script src="./js/services.js"></script>
<script src="./js/materialsController.js"></script>
</div>
</body>
</html> | {'content_hash': '8a907f4ec57286c5b0a67ad31b922539', 'timestamp': '', 'source': 'github', 'line_count': 96, 'max_line_length': 107, 'avg_line_length': 32.65625, 'alnum_prop': 0.5945773524720893, 'repo_name': 'vdtodorov93/StorageMerindjei', 'id': 'cf310c486cd1788cea282bfda8572264550f8732', 'size': '3144', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'StorageMerindjei/src/main/webapp/WEB-INF/html/materials.html', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '20'}, {'name': 'HTML', 'bytes': '16352'}, {'name': 'Java', 'bytes': '38555'}, {'name': 'JavaScript', 'bytes': '4647'}]} |
@interface ViewController : UIViewController
@end
| {'content_hash': '6dd911f11d8090c8baf3802038f0b3ca', 'timestamp': '', 'source': 'github', 'line_count': 5, 'max_line_length': 44, 'avg_line_length': 10.6, 'alnum_prop': 0.7924528301886793, 'repo_name': 'XiaoChenYung/BaiduMapTest', 'id': 'dbd149e20fed8ca58701c3d1836f0d2347fd4f3d', 'size': '220', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'BaiduMapTest/BaiduMapTest/ViewController.h', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Objective-C', 'bytes': '3676'}, {'name': 'Objective-C++', 'bytes': '1127'}]} |
class DropBillAmountAddVegeLateOptions < ActiveRecord::Migration[5.0]
def change
remove_column :bills, :amount
add_column :meal_residents, :vegetarian, :boolean, null: false, default: false
add_column :meal_residents, :late, :boolean, null: false, default: false
add_column :guests, :vegetarian, :boolean, null: false, default: false
add_column :guests, :late, :boolean, null: false, default: false
end
end
| {'content_hash': '350a901f7e50e0e0c28bfb15666d68c0', 'timestamp': '', 'source': 'github', 'line_count': 9, 'max_line_length': 82, 'avg_line_length': 47.888888888888886, 'alnum_prop': 0.7238979118329466, 'repo_name': 'joyvuu-dave/comeals', 'id': 'f61a0402a7e7f3e6d03895b05f28a94bbf3f8540', 'size': '431', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'db/migrate/20160301173036_drop_bill_amount_add_vege_late_options.rb', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '814'}, {'name': 'CoffeeScript', 'bytes': '1939'}, {'name': 'HTML', 'bytes': '33223'}, {'name': 'JavaScript', 'bytes': '6315'}, {'name': 'Ruby', 'bytes': '125071'}]} |
<div class="register-box-body">
<div class="alert alert-success" style="display:none" id="stripe_status">
Ok! Now please register!
</div>
<form action="" method="POST" id="payment-form">
<input type="hidden" name="_token" value="{{ csrf_token() }}">
<span class="payment-errors"></span>
<p class="login-box-msg"> Bank Account Data</p>
<div class="form-row form-group has-feedback">
<label>
<input class="form-control" placeholder="Card Number" type="text" size="20" data-stripe="number"/>
</label>
</div>
<div class="form-row form-group has-feedback">
<label>
<input class="form-control" placeholder="CVC" type="text" size="4" data-stripe="cvc"/>
</label>
</div>
<div class="form-row form-group has-feedback">
<label>
<input placeholder="MM" type="text" size="2" data-stripe="exp-month"/>
</label>
<span> / </span>
<input placeholder="YYYY" type="text" size="4" data-stripe="exp-year"/>
</div>
<button type="submit" class="btn btn-primary btn-block btn-flat">Submit Payment</button>
</form>
</div> | {'content_hash': '2c80f8b08ea027ae1c2cee9a4b0a1fff', 'timestamp': '', 'source': 'github', 'line_count': 32, 'max_line_length': 114, 'avg_line_length': 39.03125, 'alnum_prop': 0.5516413130504404, 'repo_name': 'alexbonavila/MySaas', 'id': 'd6054fa1ba1b33221079ee75b9d80c936be970d9', 'size': '1249', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'resources/views/auth/partials/stripe.blade.php', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'ApacheConf', 'bytes': '553'}, {'name': 'CSS', 'bytes': '2259'}, {'name': 'HTML', 'bytes': '1698308'}, {'name': 'JavaScript', 'bytes': '2834564'}, {'name': 'PHP', 'bytes': '466198'}]} |
import subprocess, signal, sys, os
from datetime import datetime
def ctrlc(sig, frame):
sys.exit(0)
signal.signal(signal.SIGINT, ctrlc)
timestamp = datetime.now().strftime('%Y%m%d-%H%M%S')
exe = './bin/benchmark/fenwick/tofile'
dst = './benchout/fenwick/{}/'.format(timestamp)
queries = '1000000'
#points = [ 2**k - 1 + 2**(k-m) - 1 for k in range(33) for m in range(k) ]
points = list(sum([ (2**k-1, 3*2**k-1) for k in range(33) for m in range(k) ], ()))
points = filter(lambda n: n > 10**5 and n < 4*10**9, points)
points = [ str(i) for i in sorted(list(set(points))) ]
os.makedirs(dst, exist_ok=True)
for size in points:
cmd = subprocess.run([ exe, dst, size, queries], stdout=sys.stdout, stderr=sys.stderr)
| {'content_hash': 'd2f4a5dd1fbe7c8c4bca8bcbbee1607b', 'timestamp': '', 'source': 'github', 'line_count': 22, 'max_line_length': 90, 'avg_line_length': 32.95454545454545, 'alnum_prop': 0.6510344827586206, 'repo_name': 'pacman616/fenwick_tree', 'id': '6bc3f6d8e66ab9563e3add2e7ffcc35a3c7dc6b7', 'size': '744', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'fenbench.sh', 'mode': '33261', 'license': 'mit', 'language': [{'name': 'C++', 'bytes': '455736'}, {'name': 'Makefile', 'bytes': '4327'}, {'name': 'Python', 'bytes': '19805'}, {'name': 'Shell', 'bytes': '620'}]} |
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"
>
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.vas</groupId>
<artifactId>vas</artifactId>
<version>1.0-GA</version>
</parent>
<artifactId>vas-opendata-paris-proxy</artifactId>
<dependencies>
<dependency>
<groupId>${project.groupId}</groupId>
<artifactId>vas-commons</artifactId>
<version>${project.version}</version>
</dependency>
<dependency>
<groupId>${project.groupId}</groupId>
<artifactId>vas-inject</artifactId>
<version>${project.version}</version>
</dependency>
<dependency>
<groupId>${project.groupId}</groupId>
<artifactId>vas-opendata-paris-client</artifactId>
<version>${project.version}</version>
</dependency>
</dependencies>
</project> | {'content_hash': '4371797f96695fbf2ad72bca8e5c5663', 'timestamp': '', 'source': 'github', 'line_count': 29, 'max_line_length': 104, 'avg_line_length': 33.48275862068966, 'alnum_prop': 0.670442842430484, 'repo_name': 'vincent7894/vas', 'id': '2a7ca0195ee1a5db95084b8e4098c3175ba11093', 'size': '971', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'vas-opendata-paris-proxy/pom.xml', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Java', 'bytes': '412736'}, {'name': 'Smarty', 'bytes': '574'}]} |
import re
import sys
import os.path
def getfilenames(base, platform):
target = re.compile('target name="([^"]+)"')
fileset = re.compile('fileset.*SRC_DIR.*includes="([^"]+)"')
# only support rhino right now
buildrhino = (platform == 'rhino')
files = []
isrhino = False
f = open(os.path.join(base, 'build.xml'))
for line in f:
# mini-sax... if we see a <target ...>
# check that it is not rhino stuff
# if so, we'll skip al the filesets
mo = target.search(line)
if mo:
fname = mo.group(1)
isrhino = (fname == 'rhino-env')
mo = fileset.search(line)
if mo and (buildrhino or not isrhino):
fname = mo.group(1)
files.append(fname)
f.close()
return files
def concat(base, src, outfile, atline=True, platform=None):
files = getfilenames(base, platform)
outfile = open(outfile, 'w')
for f in files:
fname = os.path.join(base, src, f)
data = open(fname, 'r')
if atline:
if f != 'common/outro.js' and f != 'common/intro.js':
outfile.write('\n//@line 1 "' + f + '"\n')
outfile.write(data.read())
else:
outfile.write(data.read())
data.close()
if __name__ == '__main__':
from optparse import OptionParser
parser = OptionParser()
parser.add_option('-t', '--top', dest='top', default='.',
help="Location of env-js source tree")
parser.add_option('-s', '--src', dest='src', default='src',
help="Source directory, default is 'src'")
parser.add_option('-d', '--dist', dest='dist', default='dist',
help="Destination directory, default 'dist'")
parser.add_option('-p', '--platform', dest='platform', default='',
help="platform type, optional. Defaults to core platform. 'rhino' is the only value supported")
parser.add_option('-a', '--atline', dest='atline', action="store_true",
default=False,
help="platform type, optional. Defaults to core platform. 'rhino' is the only value supported")
(options, args) = parser.parse_args()
outname = 'env.js'
if options.platform == 'rhino':
outname = 'env.rhino.js'
outfile = os.path.join(options.top, options.dist, outname)
concat(os.path.expanduser(options.top),
os.path.expanduser(options.src),
os.path.expanduser(outfile),
atline=options.atline,
platform=options.platform)
| {'content_hash': 'bbf681f204ef25b26ee11156a801dd92', 'timestamp': '', 'source': 'github', 'line_count': 72, 'max_line_length': 117, 'avg_line_length': 36.013888888888886, 'alnum_prop': 0.559583494022368, 'repo_name': 'petersibley/jsblit', 'id': '5195149ffc1f1685ad9d3871d502ae408f628f0f', 'size': '2734', 'binary': False, 'copies': '2', 'ref': 'refs/heads/master', 'path': 'tools/env-js/misc/build.py', 'mode': '33261', 'license': 'bsd-3-clause', 'language': [{'name': 'C#', 'bytes': '6597'}, {'name': 'Java', 'bytes': '71526'}, {'name': 'JavaScript', 'bytes': '2645655'}, {'name': 'Python', 'bytes': '4748'}, {'name': 'Shell', 'bytes': '12891'}]} |
default['magento']['version'] = '2.1.7'
default['magento']['edition'] = 'Community'
default['magento']['composer_project'] = 'magento/product-community-edition'
default['magento']['domain'] = 'example.com'
default['magento']['additional_domains'] = {}
# Docroot
default['magento']['docroot'] = "/var/www/#{node['magento']['domain']}"
default['magento']['installation_path'] = "#{node['magento']['docroot']}/releases/primary/magento"
default['magento']['release'] = "#{node['magento']['docroot']}/releases/primary"
# Users and groups
default['magento']['cli_user']['name'] = 'mage-cli'
default['magento']['cli_user']['group'] = 'cli'
default['magento']['www_user']['name'] = 'www-data'
default['magento']['www_user']['group'] = 'www-data'
default['magento']['update_permissions'] = true
default['magento']['directory']['permissions'] = 0775
# Installation Parameters
default['magento']['installation']['admin_first'] = 'Admin'
default['magento']['installation']['admin_last'] = 'User'
default['magento']['installation']['admin_email'] = '[email protected]'
default['magento']['installation']['admin_user'] = 'admin'
default['magento']['installation']['admin_pass'] = 'mage123'
default['magento']['installation']['language'] = 'en_US'
default['magento']['installation']['currency'] = 'USD'
default['magento']['installation']['app_timezone'] = 'America/Los_Angeles'
default['magento']['installation']['backend_frontname'] = 'admin'
default['magento']['installation']['crypt_key'] = ''
default['magento']['installation']['date'] = Time.now.strftime("%a, %d %B %Y %H:%M:%S +0000")
default['magento']['installation']['sample_data'] = false
default['magento']['installation']['verbosity'] = 'vvv'
# Session
default['magento']['session']['save'] = 'redis'
default['magento']['session']['redis']['host'] = 'localhost'
default['magento']['session']['redis']['port'] = '6379'
default['magento']['session']['redis']['password'] = ''
default['magento']['session']['redis']['timeout'] = '2.5'
default['magento']['session']['redis']['persistent_identifier'] = ''
default['magento']['session']['redis']['database'] = '0'
default['magento']['session']['redis']['compression_threshold'] = '2048'
default['magento']['session']['redis']['compression_library'] = 'gzip'
default['magento']['session']['redis']['log_level'] = '1'
default['magento']['session']['redis']['max_concurrency'] = '6'
default['magento']['session']['redis']['break_after_frontend'] = '5'
default['magento']['session']['redis']['break_after_adminhtml'] = '30'
default['magento']['session']['redis']['first_lifetime'] = '600'
default['magento']['session']['redis']['bot_first_lifetime'] = '60'
default['magento']['session']['redis']['bot_lifetime'] = '7200'
default['magento']['session']['redis']['disable_locking'] = '0'
default['magento']['session']['redis']['min_lifetime'] = '60'
default['magento']['session']['redis']['max_lifetime'] = '2592000'
# Cache
default['magento']['cache']['save'] = 'redis'
default['magento']['cache']['redis']['default']['backend'] = 'Cm_Cache_Backend_Redis'
default['magento']['cache']['redis']['default']['server'] = 'localhost'
default['magento']['cache']['redis']['default']['port'] = '6379'
default['magento']['cache']['redis']['default']['persistent'] = ''
default['magento']['cache']['redis']['default']['database'] = '1'
default['magento']['cache']['redis']['default']['password'] = ''
default['magento']['cache']['redis']['default']['force_standalone'] = '0'
default['magento']['cache']['redis']['default']['connect_retries'] = '1'
default['magento']['cache']['redis']['default']['read_timeout'] = '10'
default['magento']['cache']['redis']['default']['automatic_cleaning_factor'] = '0'
default['magento']['cache']['redis']['default']['compress_data'] = '1'
default['magento']['cache']['redis']['default']['compress_tags'] = '1'
default['magento']['cache']['redis']['default']['compress_threshold'] = '20480'
default['magento']['cache']['redis']['default']['compression_lib'] = 'gzip'
default['magento']['cache']['redis']['default']['use_lua'] = '0'
default['magento']['cache']['redis']['page_cache']['backend'] = 'Cm_Cache_Backend_Redis'
default['magento']['cache']['redis']['page_cache']['server'] = 'localhost'
default['magento']['cache']['redis']['page_cache']['port'] = '6379'
default['magento']['cache']['redis']['page_cache']['persistent'] = ''
default['magento']['cache']['redis']['page_cache']['database'] = '2'
default['magento']['cache']['redis']['page_cache']['password'] = ''
default['magento']['cache']['redis']['page_cache']['force_standalone'] = '0'
default['magento']['cache']['redis']['page_cache']['connect_retries'] = '1'
default['magento']['cache']['redis']['page_cache']['lifetimelimit'] = '57600'
default['magento']['cache']['redis']['page_cache']['compress_data'] = '0'
default['magento']['application']['php_fpm_pool'] = 'www'
default['magento']['mysql']['table_prefix'] = ''
default['magento']['mysql']['connection']['default']['dbname'] = 'magento'
default['magento']['mysql']['connection']['default']['username'] = 'mage'
default['magento']['mysql']['connection']['default']['host'] = '127.0.0.1'
default['magento']['mysql']['connection']['default']['model'] = 'mysql4'
default['magento']['mysql']['connection']['default']['engine'] = 'innodb'
default['magento']['mysql']['connection']['default']['initStatements'] = 'SET NAMES utf8'
default['magento']['mysql']['connection']['default']['active'] = '1'
default['magento']['resource']['default_setup']['connection'] = 'default'
default['magento']['x_frame_options'] = 'SAMEORIGIN'
default['magento']['mage_mode'] = 'production'
default['magento']['cache_types'] = {
"config" => 1,
"layout" => 1,
"block_html" => 1,
"collections" => 1,
"reflection" => 1,
"db_ddl" => 1,
"eav" => 1,
"customer_notification" => 1,
"full_page" => 1,
"config_integration" => 1,
"config_integration_api" => 1,
"translate" => 1,
"config_webservice" => 1
}
if node.recipe?('cop_varnish::default')
default['magento']['varnish']['enabled'] = true
else
default['magento']['varnish']['enabled'] = false
end
# n98-magerun2 attributes
default['magento']['n98']['enabled'] = true
default['magento']['n98']['version'] = '1.6.0'
default['magento']['n98']['checksum'] = '3f185a4199d0137bc0961ed6eebe37b1c9363496101df56457ddfb591523ac6a'
default['magento']['n98']['path'] = '/usr/local/bin/n98-magerun2'
default['magento']['n98']['owner'] = 'root'
default['magento']['n98']['group'] = 'root'
default['magento']['n98']['mode'] = '0755'
| {'content_hash': 'b787f5ddbf559974ae6061af8961e40d', 'timestamp': '', 'source': 'github', 'line_count': 134, 'max_line_length': 106, 'avg_line_length': 54.485074626865675, 'alnum_prop': 0.5747157923572114, 'repo_name': 'copious-cookbooks/magento', 'id': '8cbf4c2f93c828f599d6559a8b8f821e9ac81524', 'size': '7311', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'attributes/magento-default.rb', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'HTML', 'bytes': '13627'}, {'name': 'Ruby', 'bytes': '28396'}]} |
Gearpump is a lightweight real-time big data streaming engine. It is inspired by recent advances in the [Akka](https://github.com/akka/akka) framework and a desire to improve on existing streaming frameworks.
The name Gearpump is a reference to the engineering term "gear pump", which is a super simple pump that consists of only two gears, but is very powerful at streaming water.

We model streaming within the Akka actor hierarchy.

Per initial benchmarks we are able to process near 18 million messages/second (100 bytes per message) with a 8ms latency on a 4-node cluster.
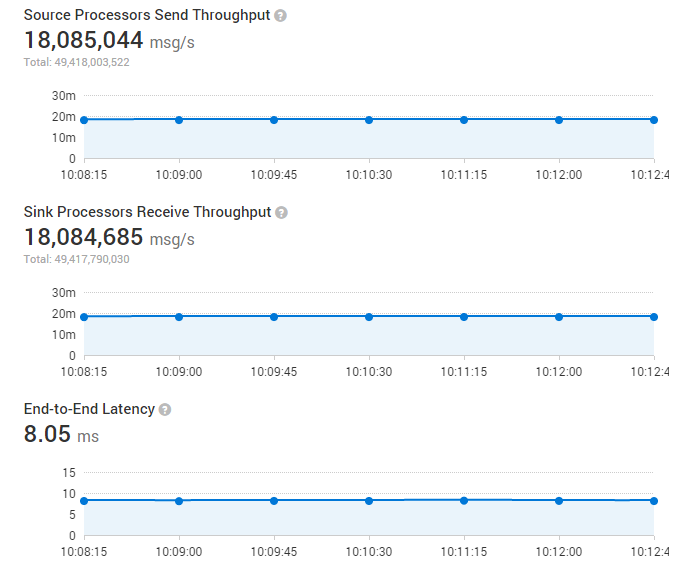
For steps to reproduce the performance test, please check [Performance benchmark](http://gearpump.apache.org/releases/latest/introduction/performance-report/index.html).
## Useful Resources
* Read the [Introduction on TypeSafe's Blog](https://typesafe.com/blog/gearpump-real-time-streaming-engine-using-akka)
* Learn the [Basic Concepts](http://gearpump.apache.org/releases/latest/introduction/basic-concepts/index.html)
* How to [Develop your first application](http://gearpump.apache.org/releases/latest/dev/dev-write-1st-app/index.html)
* How to [Submit your first application](http://gearpump.apache.org/releases/latest/introduction/submit-your-1st-application/index.html)
* Explore the [Maven dependencies](http://gearpump.apache.org/downloads.html#maven-dependencies)
* Explore the [Document site](http://gearpump.apache.org)
* Explore the [User Mailing List](http://mail-archives.apache.org/mod_mbox/incubator-gearpump-user/)
* Report an [issue](https://issues.apache.org/jira/browse/GEARPUMP)
## How to Build
1). Clone the Gearpump repository
```bash
git clone https://github.com/apache/incubator-gearpump.git
cd gearpump
```
2). Build package
```bash
## Please use scala 2.11 or 2.10
## The target package path: output/target/gearpump-${version}.zip
sbt clean +assembly +packArchiveZip
```
After the build, there will be a package file gearpump-${version}.zip generated under output/target/ folder.
To build scala document, use
```bash
## Will generate the scala doc under target/scala_2.xx/unidoc/
sbt unidoc
```
**NOTE:**
The build requires network connection. If you are behind an enterprise proxy, make sure you have set the proxy in your env before running the build commands.
For windows:
```bash
set HTTP_PROXY=http://host:port
set HTTPS_PROXY= http://host:port
```
For Linux:
```bash
export HTTP_PROXY=http://host:port
export HTTPS_PROXY=http://host:port
```
## How to run Gearpump integration test
Gearpump has an integration test system which is based on Docker. Please check [the instructions](integrationtest/README.md).
## How to do style check before submitting a pull request?
Before submitting a PR, you should always run style check first:
```
## Run style check for compile, test, and integration test.
sbt scalastyle test:scalastyle it:scalastyle
```
## How to generate the license report to generate a list of all dependencies
```
sbt dumpLicenseReport
```
## How to generate dependencies by declared license
```
sbt dependencyLicenseInfo
```
## Contributors (time order)
* [Sean Zhong](https://github.com/clockfly)
* [Kam Kasravi](https://github.com/kkasravi)
* [Manu Zhang](https://github.com/manuzhang)
* [Huafeng Wang](https://github.com/huafengw)
* [Weihua Jiang](https://github.com/whjiang)
* [Suneel Marthi](https://github.com/smarthi)
* [Stanley Xu](https://github.com/stanleyxu2005)
* [Tomasz Targonski](https://github.com/TomaszT)
* [Sun Kewei](https://github.com/skw1992)
* [Gong Yu](https://github.com/pangolulu)
* [Karol Brejna](https://github.com/karol-brejna-i)
## Contacts
* [Subscribe](mailto:[email protected]) or [mail](mailto:[email protected]) the [[email protected]](http://mail-archives.apache.org/mod_mbox/incubator-gearpump-user/) list
* [Subscribe](mailto:[email protected]) or [mail](mailto:[email protected]) the [[email protected]](http://mail-archives.apache.org/mod_mbox/incubator-gearpump-dev/) list
* Report issues on [JIRA](https://issues.apache.org/jira/browse/GEARPUMP)
## License
Gearpump itself is licensed under the [Apache License (2.0)](http://www.apache.org/licenses/LICENSE-2.0).
For library it used, please see [LICENSE](https://github.com/apache/incubator-gearpump/blob/master/LICENSE).
## Acknowledgement
The netty transport code work is based on [Apache Storm](http://storm.apache.org). Thanks Apache Storm contributors.
The cgroup code work is based on [JStorm](https://github.com/alibaba/jstorm). Thanks JStorm contributors.
Thanks to Jetbrains for providing a [IntelliJ IDEA Free Open Source License](https://www.jetbrains.com/buy/opensource/?product=idea).
| {'content_hash': '007ea408776bd4a73b818e57a4545b4a', 'timestamp': '', 'source': 'github', 'line_count': 121, 'max_line_length': 233, 'avg_line_length': 41.85950413223141, 'alnum_prop': 0.7618953603158933, 'repo_name': 'manuzhang/incubator-gearpump', 'id': 'ac1c8172212deeae0809b3c722a2916057198243', 'size': '5718', 'binary': False, 'copies': '2', 'ref': 'refs/heads/master', 'path': 'README.md', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'CSS', 'bytes': '3375'}, {'name': 'Dockerfile', 'bytes': '7205'}, {'name': 'HTML', 'bytes': '75506'}, {'name': 'Java', 'bytes': '184587'}, {'name': 'JavaScript', 'bytes': '201315'}, {'name': 'Python', 'bytes': '14550'}, {'name': 'Scala', 'bytes': '1752501'}, {'name': 'Shell', 'bytes': '30874'}]} |
@class NSArray, NSDictionary, NSSet, NSString, XCAccessibilityElement, XCElementSnapshot, XCTTimeoutControls;
@protocol XCTElementSnapshotAttributeDataSource, XCTElementSnapshotProvider;
@interface XCTElementQuery : NSObject <NSSecureCoding, XCTCapabilitiesProviding>
{
_Bool _isMacOS;
_Bool _suppressAttributeKeyPathAnalysis;
_Bool _useLegacyElementType;
XCAccessibilityElement *_rootElement;
unsigned long long _options;
XCTTimeoutControls *_timeoutControls;
id <XCTElementSnapshotProvider> _snapshotProvider;
id <XCTElementSnapshotAttributeDataSource> _elementSnapshotAttributeDataSource;
XCElementSnapshot *_rootElementSnapshot;
CDUnknownBlockType _evaluationContext;
NSArray *_transformers;
}
+ (id)_firstMatchTransformerSubarraysFromArray:(id)arg1 trailingMatchAllTransformers:(id *)arg2;
+ (void)provideCapabilitiesToBuilder:(id)arg1;
+ (id)_descriptionForTransformerArray:(id)arg1;
+ (_Bool)supportsSecureCoding;
- (void).cxx_destruct;
@property _Bool useLegacyElementType; // @synthesize useLegacyElementType=_useLegacyElementType;
@property(readonly, copy) NSArray *transformers; // @synthesize transformers=_transformers;
@property(copy, nonatomic) CDUnknownBlockType evaluationContext; // @synthesize evaluationContext=_evaluationContext;
@property(retain) XCElementSnapshot *rootElementSnapshot; // @synthesize rootElementSnapshot=_rootElementSnapshot;
@property _Bool suppressAttributeKeyPathAnalysis; // @synthesize suppressAttributeKeyPathAnalysis=_suppressAttributeKeyPathAnalysis;
@property __weak id <XCTElementSnapshotAttributeDataSource> elementSnapshotAttributeDataSource; // @synthesize elementSnapshotAttributeDataSource=_elementSnapshotAttributeDataSource;
@property(retain) id <XCTElementSnapshotProvider> snapshotProvider; // @synthesize snapshotProvider=_snapshotProvider;
@property(retain) XCTTimeoutControls *timeoutControls; // @synthesize timeoutControls=_timeoutControls;
@property(readonly) _Bool isMacOS; // @synthesize isMacOS=_isMacOS;
@property(readonly) unsigned long long options; // @synthesize options=_options;
@property(readonly, copy) XCAccessibilityElement *rootElement; // @synthesize rootElement=_rootElement;
- (id)_allMatchingSnapshotsForInput:(id)arg1 transformers:(id)arg2 relatedElements:(id *)arg3 noMatchesMessage:(id *)arg4 error:(id *)arg5;
- (id)_firstMatchingSnapshotForInput:(id)arg1 transformers:(id)arg2 relatedElements:(id *)arg3 noMatchesMessage:(id *)arg4 error:(id *)arg5;
- (id)_firstMatchingSnapshotForInput:(id)arg1 transformersSubarrays:(id)arg2 relatedElements:(id *)arg3 noMatchesMessage:(id *)arg4 error:(id *)arg5;
- (id)matchingSnapshotsInSnapshotTree:(id)arg1 relatedElements:(id *)arg2 noMatchesMessage:(id *)arg3 error:(id *)arg4;
- (id)matchingSnapshotsWithRelatedElements:(id *)arg1 noMatchesMessage:(id *)arg2 error:(id *)arg3;
- (id)_snapshotForElement:(id)arg1 error:(id *)arg2;
- (id)_rootElementSnapshot:(id *)arg1;
@property(readonly, copy) NSDictionary *snapshotParameters;
@property(readonly, copy) NSArray *snapshotAttributes;
@property(readonly, copy) NSSet *elementTypes;
- (_Bool)hasTransformerWithStopsOnFirstMatch;
@property(readonly) _Bool supportsAttributeKeyPathAnalysis;
- (_Bool)canBeRemotelyEvaluatedWithCapabilities:(id)arg1;
@property(readonly, copy) NSString *description;
- (_Bool)isEqual:(id)arg1;
@property(readonly) unsigned long long hash;
- (void)encodeWithCoder:(id)arg1;
- (id)initWithCoder:(id)arg1;
- (id)initWithRootElement:(id)arg1 transformers:(id)arg2 options:(unsigned long long)arg3 isMacOS:(_Bool)arg4 timeoutControls:(id)arg5;
- (id)initWithRootElement:(id)arg1 transformers:(id)arg2 options:(unsigned long long)arg3 isMacOS:(_Bool)arg4;
- (id)initWithRootElement:(id)arg1 transformers:(id)arg2 options:(unsigned long long)arg3;
- (id)initWithRootElement:(id)arg1 transformers:(id)arg2;
// Remaining properties
@property(readonly, copy) NSString *debugDescription;
@property(readonly) Class superclass;
@end
| {'content_hash': '9048e6b7476bf70597590571eaa5e872', 'timestamp': '', 'source': 'github', 'line_count': 63, 'max_line_length': 182, 'avg_line_length': 63.142857142857146, 'alnum_prop': 0.8056812468577175, 'repo_name': 'linkedin/bluepill', 'id': 'd9762739818dd961e5099d93672881696cc07edd', 'size': '4273', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'bp/src/PrivateHeaders/XCTAutomationSupport/XCTElementQuery.h', 'mode': '33188', 'license': 'bsd-2-clause', 'language': [{'name': 'C', 'bytes': '3119'}, {'name': 'HTML', 'bytes': '13423'}, {'name': 'Objective-C', 'bytes': '820220'}, {'name': 'Python', 'bytes': '6253'}, {'name': 'Ruby', 'bytes': '689'}, {'name': 'Shell', 'bytes': '7389'}, {'name': 'Starlark', 'bytes': '5932'}, {'name': 'Swift', 'bytes': '666'}]} |
class QueryResultSumTable : public QWidget
{
Q_OBJECT
private:
QStandardItemModel* tableModel;
QTableView* tableView;
QAction* transposeAction;
QAction* saveToFileAction;
public:
explicit QueryResultSumTable(QWidget *parent = 0);
void pivot(QSqlQueryModel* model,
QString rowFieldName,
QString columnFieldName,
QString valueFieldName);
public slots:
void transpose();
void save();
signals:
public slots:
};
#endif // QUERYRESULTSUMTABLE_H
| {'content_hash': '8b0d3db812ac83c2c01fcafbbe348746', 'timestamp': '', 'source': 'github', 'line_count': 23, 'max_line_length': 54, 'avg_line_length': 22.695652173913043, 'alnum_prop': 0.6800766283524904, 'repo_name': 'AlexanderMuzalev/WebLogAnalizer', 'id': '0f0eeb57e36d33830354a647ab522a1126070ea3', 'size': '700', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/queryresultsumtable.h', 'mode': '33188', 'license': 'bsd-2-clause', 'language': [{'name': 'C', 'bytes': '7134356'}, {'name': 'C++', 'bytes': '1793690'}, {'name': 'Inno Setup', 'bytes': '7156'}, {'name': 'QML', 'bytes': '4021'}, {'name': 'QMake', 'bytes': '3375'}]} |
/**
*
*/
package me.learn.personal.month4;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.TreeMap;
import java.util.stream.Collectors;
/**
* Title 1152 :
*
* We are given some website visits: the user with name username[i] visited the
* website website[i] at time timestamp[i].
*
* A 3-sequence is a list of websites of length 3 sorted in ascending order by
* the time of their visits. (The websites in a 3-sequence are not necessarily
* distinct.)
*
* Find the 3-sequence visited by the largest number of users. If there is more
* than one solution, return the lexicographically smallest such 3-sequence.
*
*
*
* Example 1:
*
* Input: username =
* ["joe","joe","joe","james","james","james","james","mary","mary","mary"],
* timestamp = [1,2,3,4,5,6,7,8,9,10], website =
* ["home","about","career","home","cart","maps","home","home","about","career"]
* Output: ["home","about","career"] Explanation: The tuples in this example
* are: ["joe", 1, "home"] ["joe", 2, "about"] ["joe", 3, "career"] ["james", 4,
* "home"] ["james", 5, "cart"] ["james", 6, "maps"] ["james", 7, "home"]
* ["mary", 8, "home"] ["mary", 9, "about"] ["mary", 10, "career"] The
* 3-sequence ("home", "about", "career") was visited at least once by 2 users.
* The 3-sequence ("home", "cart", "maps") was visited at least once by 1 user.
* The 3-sequence ("home", "cart", "home") was visited at least once by 1 user.
* The 3-sequence ("home", "maps", "home") was visited at least once by 1 user.
* The 3-sequence ("cart", "maps", "home") was visited at least once by 1 user.
*
* @author bramanarayan
* @date Aug 22, 2020
*/
public class AnalyzeUserWebsiteVisitPattern {
/**
* @param args
*/
public static void main(String[] args) {
AnalyzeUserWebsiteVisitPattern solution = new AnalyzeUserWebsiteVisitPattern();
/*
* String[] users = new String[]
* {"joe","joe","joe","james","james","james","james","mary","mary","mary"};
* String[] pages = new String[]
* {"home","about","career","home","cart","maps","home","home","about","career"}
* ; int[] time = new int[] {1,2,3,4,5,6,7,8,9,10};
*/
String[] users = new String[] { "u1", "u1", "u1", "u2", "u2", "u2" };
String[] pages = new String[] { "a", "b", "c", "a", "b", "a" };
int[] time = new int[] { 1, 2, 3, 4, 5, 6 };
/**
* ["u1","u1","u1","u2","u2","u2"] [1,2,3,4,5,6] ["a","b","c","a","b","a"]
*/
solution.mostVisitedPattern(users, time, pages);
}
class Visit {
String userName;
int timestamp;
String website;
Visit(String u, int t, String w) {
userName = u;
timestamp = t;
website = w;
}
Visit() {
}
}
// Entry point
public List<String> mostVisitedPattern(String[] username, int[] timestamp, String[] website) {
// Convert all the entry as visit object to ease of understand
List<Visit> visitList = new ArrayList<>();
for (int i = 0; i < username.length; i++) {
visitList.add(new Visit(username[i], timestamp[i], website[i]));
}
// Sort all the visit entries using their timestamp -> ADOPT IT !!!!
Comparator<Visit> cmp = (v1, v2) -> {
return v1.timestamp - v2.timestamp;
};
Collections.sort(visitList, cmp);
// Collect list of websites for each user
Map<String, List<String>> userWebSitesMap = new HashMap<>();
for (Visit v : visitList) {
userWebSitesMap.putIfAbsent(v.userName, new ArrayList<>());
userWebSitesMap.get(v.userName).add(v.website);
}
Map<List<String>, Integer> seqUserFreMap = new HashMap<>();
// Now get all the values of all the users
for (List<String> websitesList : userWebSitesMap.values()) {
if (websitesList.size() < 3)
continue; // no need to consider less than 3 entries of web site visited by user
// if its more than or equal to 3.
Set<List<String>> sequencesSet = generate3Seq(websitesList);
// Now update the frequency of the sequence ( increment by 1 for 1 user)
for (List<String> seq : sequencesSet) {
seqUserFreMap.putIfAbsent(seq, 0);
seqUserFreMap.put(seq, seqUserFreMap.get(seq) + 1);
}
}
List<String> res = new ArrayList<>();
int MAX = 0;
for (Map.Entry<List<String>, Integer> entry : seqUserFreMap.entrySet()) {
if (entry.getValue() > MAX) {
MAX = entry.getValue();
res = entry.getKey();
} else if (entry.getValue() == MAX) {
// for lexicographic sort requirement
if (entry.getKey().toString().compareTo(res.toString()) < 0) {
res = entry.getKey();
}
}
}
return res;
}
// It will not return duplicate seq for each user that why we are using Set
private Set<List<String>> generate3Seq(List<String> websitesList) {
Set<List<String>> setOfListSeq = new HashSet<>();
for (int i = 0; i < websitesList.size(); i++) {
for (int j = i + 1; j < websitesList.size(); j++) {
for (int k = j + 1; k < websitesList.size(); k++) {
List<String> list = new ArrayList<>();
list.add(websitesList.get(i));
list.add(websitesList.get(j));
list.add(websitesList.get(k));
setOfListSeq.add(list);
}
}
}
return setOfListSeq;
}
// MY sol
public List<String> mostVisitedPatternMy(String[] username, int[] timestamp, String[] website) {
// user -> pages visited
Map<String, List<String>> map = new HashMap<>();
// pattern 3 tuple -> count
TreeMap<String, Integer> count = new TreeMap<>();
int n = username.length;
for (int i = 0; i < n; i++) {
String user = username[i];
String page = website[i];
int time = timestamp[i];
map.computeIfAbsent(user, x -> new ArrayList<String>()).add(page);
if (map.get(user).size() >= 3) {
String pattern = get3Tuple(map.get(user));
count.put(pattern, count.getOrDefault(pattern, 0) + 1);
}
}
int max = Collections.max(count.values());
List<String> resultSet = count.entrySet().stream().filter(e -> e.getValue() == max).map(e -> e.getKey())
.collect(Collectors.toList());
Collections.sort(resultSet);
String maxPattern = resultSet.get(0);
String[] websites = maxPattern.split("#");
List<String> result = new ArrayList<String>();
result.add(websites[0]);
result.add(websites[1]);
result.add(websites[2]);
return result;
}
private String get3Tuple(List<String> list) {
int n = list.size();
return String.format("%s#%s#%s", list.get(n - 3), list.get(n - 2), list.get(n - 1));
}
}
| {'content_hash': 'd6092b58d68ce750d12719ea54b9ab0d', 'timestamp': '', 'source': 'github', 'line_count': 209, 'max_line_length': 106, 'avg_line_length': 31.124401913875598, 'alnum_prop': 0.6304381245196004, 'repo_name': 'balajiboggaram/algorithms', 'id': '188929d19e069e6064e549478d1b0df341765c99', 'size': '6505', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/me/learn/personal/month4/AnalyzeUserWebsiteVisitPattern.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Java', 'bytes': '20090'}]} |
package com.mycompany.ibbasics;
import org.robovm.apple.uikit.UILabel;
import org.robovm.apple.uikit.UITextField;
import org.robovm.apple.uikit.UIViewController;
import org.robovm.objc.annotation.CustomClass;
import org.robovm.objc.annotation.IBAction;
import org.robovm.objc.annotation.IBOutlet;
@CustomClass("GreetingsController")
public class GreetingsController extends UIViewController {
private UILabel label;
private UITextField textField;
@IBOutlet
public void setLabel(UILabel label) {
this.label = label;
}
@IBOutlet
public void setTextField(UITextField textField) {
this.textField = textField;
}
@IBAction
private void clicked() {
String name = textField.getText();
if (name.isEmpty()) {
name = "Unknown";
}
label.setText("Hello " + name + "!");
}
@IBAction
private void textChanged() {
label.setText("I see you typing!");
}
}
| {'content_hash': '365e6f4b6d131b2401343c36b0fef241', 'timestamp': '', 'source': 'github', 'line_count': 38, 'max_line_length': 59, 'avg_line_length': 25.473684210526315, 'alnum_prop': 0.6766528925619835, 'repo_name': 'robovm/robovm-tutorials', 'id': '485a5e66bd21bd6a5cff2d5b26c5988f7c11a350', 'size': '968', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'ib-basics/src/main/java/com/mycompany/ibbasics/GreetingsController.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Java', 'bytes': '20710'}]} |
package dragAndDrop;
import java.awt.EventQueue;
import javax.swing.JFrame;
import javax.swing.JTextField;
import javax.swing.JButton;
import java.awt.Font;
import java.awt.Color;
import javax.swing.SwingConstants;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
public class ATM_GUI {
private JFrame frmAtmServer;
private JTextField textField;
private JTextField textField_1;
private JTextField textField_2;
private JTextField textField_3;
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
ATM_GUI window = new ATM_GUI();
window.frmAtmServer.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the application.
*/
public ATM_GUI() {
initialize();
}
/**
* Initialize the contents of the frame.
*/
private void initialize() {
frmAtmServer = new JFrame();
frmAtmServer.setTitle("ATM service item ***Made by xgqfrms***");
frmAtmServer.getContentPane().setBackground(Color.CYAN);
frmAtmServer.getContentPane().setForeground(Color.GREEN);
frmAtmServer.getContentPane().setFont(new Font("ËÎÌå", Font.BOLD, 18));
frmAtmServer.setResizable(false);
frmAtmServer.setBounds(100, 100, 656, 468);
frmAtmServer.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frmAtmServer.getContentPane().setLayout(null);
textField = new JTextField();
textField.setEditable(false);
textField.setHorizontalAlignment(SwingConstants.CENTER);
textField.setForeground(Color.GREEN);
textField.setFont(new Font("ËÎÌå", Font.BOLD, 18));
textField.setText("\u4F59\u989D\u67E5\u8BE2\uFF1A");
textField.setBounds(62, 56, 160, 42);
frmAtmServer.getContentPane().add(textField);
textField.setColumns(10);
textField_1 = new JTextField();
textField_1.setEditable(false);
textField_1.setHorizontalAlignment(SwingConstants.CENTER);
textField_1.setFont(new Font("ËÎÌå", Font.BOLD, 18));
textField_1.setForeground(Color.GREEN);
textField_1.setText("\u53D6\u6B3E\uFF1A");
textField_1.setBounds(62, 139, 160, 42);
frmAtmServer.getContentPane().add(textField_1);
textField_1.setColumns(10);
textField_2 = new JTextField();
textField_2.setEditable(false);
textField_2.setText("\u5B58\u6B3E\uFF1A");
textField_2.setHorizontalAlignment(SwingConstants.CENTER);
textField_2.setFont(new Font("ËÎÌå", Font.BOLD, 18));
textField_2.setForeground(Color.GREEN);
textField_2.setBounds(62, 223, 160, 40);
frmAtmServer.getContentPane().add(textField_2);
textField_2.setColumns(10);
textField_3 = new JTextField();
textField_3.setEditable(false);
textField_3.setText("\u4FEE\u6539\u5BC6\u7801:");
textField_3.setHorizontalAlignment(SwingConstants.CENTER);
textField_3.setForeground(Color.GREEN);
textField_3.setFont(new Font("ËÎÌå", Font.BOLD, 18));
textField_3.setBounds(62, 302, 158, 42);
frmAtmServer.getContentPane().add(textField_3);
textField_3.setColumns(10);
JButton btnNewButton = new JButton("cheek");
btnNewButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
}
});
btnNewButton.setForeground(Color.BLUE);
btnNewButton.setBackground(Color.GREEN);
btnNewButton.setFont(new Font("ËÎÌå", Font.PLAIN, 18));
btnNewButton.setBounds(294, 48, 149, 57);
frmAtmServer.getContentPane().add(btnNewButton);
JButton btnNewButton_1 = new JButton("withdraw");
btnNewButton_1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
}
});
btnNewButton_1.setBackground(Color.GREEN);
btnNewButton_1.setForeground(Color.BLUE);
btnNewButton_1.setFont(new Font("ËÎÌå", Font.PLAIN, 18));
btnNewButton_1.setBounds(294, 128, 151, 63);
frmAtmServer.getContentPane().add(btnNewButton_1);
JButton btnSave = new JButton("save");
btnSave.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
}
});
btnSave.setBackground(Color.GREEN);
btnSave.setFont(new Font("ËÎÌå", Font.PLAIN, 18));
btnSave.setForeground(Color.BLUE);
btnSave.setBounds(294, 214, 149, 57);
frmAtmServer.getContentPane().add(btnSave);
JButton btnModify = new JButton("modify");
btnModify.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
}
});
btnModify.setBackground(Color.GREEN);
btnModify.setFont(new Font("ËÎÌå", Font.PLAIN, 18));
btnModify.setForeground(Color.BLUE);
btnModify.setBounds(294, 287, 149, 63);
frmAtmServer.getContentPane().add(btnModify);
JButton btnNewButton_2 = new JButton("\u9000\u51FAATM");
btnNewButton_2.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
}
});
btnNewButton_2.setForeground(Color.BLACK);
btnNewButton_2.setFont(new Font("ËÎÌå", Font.BOLD, 18));
btnNewButton_2.setBackground(Color.RED);
btnNewButton_2.setBounds(512, 10, 116, 42);
frmAtmServer.getContentPane().add(btnNewButton_2);
}
}
| {'content_hash': '8d0dc464a3e7413fdfa66285efae383e', 'timestamp': '', 'source': 'github', 'line_count': 154, 'max_line_length': 73, 'avg_line_length': 32.64935064935065, 'alnum_prop': 0.7322991249005569, 'repo_name': 'xgqfrms/JavaWeb', 'id': 'fc3ae583ea6f1f5dc0fd23a98e7a19c96db00131', 'size': '5028', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'JavaGUI/src/dragAndDrop/ATM_GUI.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'CSS', 'bytes': '23228'}, {'name': 'HTML', 'bytes': '22801'}, {'name': 'Java', 'bytes': '868770'}, {'name': 'JavaScript', 'bytes': '490'}]} |
+++
title = "Note on Apr 19 EDT 2018 at 11:7"
publishdate = 2018-04-19T15:07:00+00:00
draft = false
syndicated = [ 'https://twitter.com/chrisjohndeluca/status/986984516345647100?s=20' ]
+++
Five years after Google conquered and abandoned RSS, the news-reader ecosystem is showing green shoots https://t.co/KmxYfqeJjC
| {'content_hash': '5e478bf5f33282a974c5d20c08438147', 'timestamp': '', 'source': 'github', 'line_count': 8, 'max_line_length': 126, 'avg_line_length': 39.75, 'alnum_prop': 0.7515723270440252, 'repo_name': 'bronzehedwick/chrisdeluca', 'id': 'cf66aac0355833b06e25c3cd97a5a70cfbfac87b', 'size': '318', 'binary': False, 'copies': '1', 'ref': 'refs/heads/main', 'path': 'content/note/1524150420000.md', 'mode': '33261', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '24896'}, {'name': 'HTML', 'bytes': '48303'}, {'name': 'JavaScript', 'bytes': '7942'}, {'name': 'Lua', 'bytes': '911'}, {'name': 'Makefile', 'bytes': '1607'}, {'name': 'PHP', 'bytes': '7829'}, {'name': 'Shell', 'bytes': '1474'}]} |
package scalaguide.common.build
package object controllers {
type AssetsBuilder = _root_.controllers.AssetsBuilder
type Assets = _root_.controllers.Assets
}
| {'content_hash': '329a1ab94875d0cfac1ffb63f237604a', 'timestamp': '', 'source': 'github', 'line_count': 8, 'max_line_length': 55, 'avg_line_length': 21.375, 'alnum_prop': 0.7485380116959064, 'repo_name': 'playframework/playframework', 'id': '06e981dcf98f05b5a7dad1acf247eb84ecacbf4c', 'size': '325', 'binary': False, 'copies': '1', 'ref': 'refs/heads/main', 'path': 'documentation/manual/working/commonGuide/build/code/scalaguide/common/build/controllers/SubProjectAssets.scala', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'CSS', 'bytes': '86'}, {'name': 'HTML', 'bytes': '44899'}, {'name': 'Java', 'bytes': '1547751'}, {'name': 'JavaScript', 'bytes': '9846'}, {'name': 'Less', 'bytes': '1577'}, {'name': 'Roff', 'bytes': '6187'}, {'name': 'Scala', 'bytes': '3648741'}]} |
import { Environment } from 'environments/environment-type';
import { testEnvironmentBase } from 'environments/test-env-base';
// This file is used in the deployed test environment
export const environment: Environment = {
...testEnvironmentBase,
allOfUsApiUrl:
'https://pr-9-dot-api-dot-all-of-us-workbench-test.appspot.com',
displayTag: 'PR Site 9',
debug: false,
};
| {'content_hash': '47146fcfefade8d3243919554d7c417f', 'timestamp': '', 'source': 'github', 'line_count': 11, 'max_line_length': 68, 'avg_line_length': 34.72727272727273, 'alnum_prop': 0.7356020942408377, 'repo_name': 'all-of-us/workbench', 'id': '9a92294f36c018de94c7808dd0ca13d78af6e6fb', 'size': '382', 'binary': False, 'copies': '1', 'ref': 'refs/heads/main', 'path': 'ui/src/environments/environment.pr-site-9.ts', 'mode': '33188', 'license': 'bsd-3-clause', 'language': [{'name': 'CSS', 'bytes': '14709'}, {'name': 'Dockerfile', 'bytes': '1601'}, {'name': 'Groovy', 'bytes': '5004'}, {'name': 'HTML', 'bytes': '354263'}, {'name': 'Java', 'bytes': '4258561'}, {'name': 'JavaScript', 'bytes': '104985'}, {'name': 'Jupyter Notebook', 'bytes': '12135'}, {'name': 'Kotlin', 'bytes': '76275'}, {'name': 'Mustache', 'bytes': '126650'}, {'name': 'Python', 'bytes': '52410'}, {'name': 'R', 'bytes': '1157'}, {'name': 'Ruby', 'bytes': '237944'}, {'name': 'Shell', 'bytes': '507090'}, {'name': 'TypeScript', 'bytes': '3656309'}, {'name': 'wdl', 'bytes': '31820'}]} |
package esteban.reminder.java6.annotations;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
/**
* This code demonstrates how to define an annotation.
*
* @author Yashwant Golecha ([email protected])
* @version 1.0
*/
@Target(ElementType.METHOD)
@Retention(RetentionPolicy.RUNTIME)
@interface Todo {
public enum Priority {LOW, MEDIUM, HIGH}
public enum Status {STARTED, NOT_STARTED}
String author() default "Yash";
Priority priority() default Priority.LOW;
Status status() default Status.NOT_STARTED;
}
| {'content_hash': 'bcb58ebc9fd674a00d9d7a7bbaa52da7', 'timestamp': '', 'source': 'github', 'line_count': 22, 'max_line_length': 54, 'avg_line_length': 29.454545454545453, 'alnum_prop': 0.7515432098765432, 'repo_name': 'estebangm81/java6_reminder', 'id': '6f3a6ee728d70441b45653c093eda929fef71185', 'size': '648', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'java6_features/src/esteban/reminder/java6/annotations/Todo.java', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Java', 'bytes': '13633'}]} |
public class Dog implements Comparable<Dog>{
String name;
int size;
public Dog(String n,int s){
this.name = n;
this.size = s;
}
public void bark(){
System.out.println(name+" bark");
}
@Override
public int compareTo(Dog AnotherDog){
return this.size - AnotherDog.size;
}
} | {'content_hash': '58e2962f7224f31126e5eb82a3699301', 'timestamp': '', 'source': 'github', 'line_count': 19, 'max_line_length': 44, 'avg_line_length': 15.578947368421053, 'alnum_prop': 0.6655405405405406, 'repo_name': 'FTJiang/cs61B-17Spring', 'id': 'c6d6aa79164572b4ddc34126ecf9ec83d4ccf7fb', 'size': '299', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'ObjCompare/comparable/Dog.java', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Batchfile', 'bytes': '3'}, {'name': 'CSS', 'bytes': '3780'}, {'name': 'HTML', 'bytes': '13033'}, {'name': 'Java', 'bytes': '195208'}, {'name': 'JavaScript', 'bytes': '5252'}]} |
import math, csv
import numpy as np
class SpatialGrid(object):
def __init__(self, x0_corner, y0_corner, sizex, sizey, ncols, nrows):
self.y0_corner = y0_corner
self.x0_corner = x0_corner
self.sizex = sizex
self.sizey = sizey
self.ncols = ncols
self.nrows = nrows
def get_config(self):
return [self.x0_corner, self.y0_corner, self.sizex, self.sizey, self.ncols, self.nrows]
def rowcol(self, latitude, longitude):
row = int(math.floor((self.y0_corner - latitude) / -self.sizey))
col = int(math.floor((longitude - self.x0_corner) / self.sizex))
return (row, col)
def getll_raw(self, latitude, longitude):
raise NotImplementedError()
def get_longitudes(self):
return np.arange(self.x0_corner + self.sizex / 2, self.x0_corner + self.sizex * self.ncols,
step=self.sizex)
def get_latitudes(self):
return np.arange(self.y0_corner + self.sizey * self.nrows, self.y0_corner + self.sizey / 2,
step=-self.sizey)
class NumpyGrid(SpatialGrid):
def __init__(self, array, x0_corner, y0_corner, sizex, sizey, ncols, nrows):
super(NumpyGrid, self).__init__(x0_corner, y0_corner, sizex, sizey, ncols, nrows)
self.array = array
@staticmethod
def from_regular_axes(array, latitude, longitude):
return NumpyGrid(array, min(longitude), max(latitude), abs(np.mean(np.diff(longitude))),
-abs(np.mean(np.diff(latitude))), array.shape[1], array.shape[0])
def getll_raw(self, latitude, longitude):
row = int(math.floor((self.y0_corner - latitude) / self.sizey))
col = int(math.floor((longitude - self.x0_corner) / self.sizex))
return self.array[row, col]
@staticmethod
def convert(grid, nvals, agfactor=1):
lons = grid.get_longitudes()
lats = grid.get_latitudes()
array = np.zeros((len(lats), len(lons), nvals))
for ii in np.arange(agfactor / 2, len(lons), agfactor):
for jj in np.arange(agfactor / 2, len(lats), agfactor):
array[jj, ii, :] = grid.getll_raw(lats[jj], lons[ii])
return NumpyGrid(array, grid.x0_corner, grid.y0_corner, grid.sizex * agfactor, grid.sizey * agfactor, grid.ncols / agfactor, grid.nrows / agfactor)
class DelimitedSpatialGrid(SpatialGrid):
def __init__(self, fp, x0_corner, y0_corner, sizex, sizey, ncols, nrows, delimiter):
"""Note that delimiter can be None, in which case multiple whitespace characters are the delimiter."""
super(DelimitedSpatialGrid, self).__init__(x0_corner, y0_corner, sizex, sizey, ncols, nrows)
if isinstance(fp, np.ndarray):
self.values = values
else:
values = []
for line in fp:
values.append(map(float, line.split(delimiter)))
self.values = np.array(values)
def getll_raw(self, latitude, longitude):
row, col = self.rowcol(latitude, longitude)
if row >= self.values.shape[0] or col >= self.values.shape[1]:
return np.nan
return self.values[row, col]
@staticmethod
def write(grid, fp, delimiter, nodataval):
"""Write to the file pointer `fp`."""
writer = csv.writer(fp, delimiter=delimiter)
for latitude in grid.get_latitudes()[::-1]:
row = []
for longitude in grid.get_longitudes():
value = grid.getll_raw(latitude, longitude)
if np.isnan(value):
row.append(nodataval)
else:
row.append(value)
writer.writerow(row)
class AsciiSpatialGrid(DelimitedSpatialGrid):
def __init__(self, fp, delimiter):
count = 0
for line in fp:
vals = line.split(delimiter)
if vals[0] == 'ncols':
ncols = int(vals[1])
if vals[0] == 'nrows':
nrows = int(vals[1])
if vals[0] == 'xllcorner':
xllcorner = float(vals[1])
if vals[0] == 'yllcorner':
yllcorner = float(vals[1])
if vals[0] == 'cellsize':
cellsize = float(vals[1])
if vals[0] == 'NODATA_value':
nodata = float(vals[1])
count += 1
if count == 6:
break
super(AsciiSpatialGrid, self).__init__(fp, xllcorner, yllcorner, cellsize, cellsize, ncols, nrows, delimiter)
self.values[self.values == nodata] = np.nan
@staticmethod
def write(grid, fp, nodataval):
"""Write to the file pointer `fp`."""
fp.write("ncols\t%d\n" % grid.ncols)
fp.write("nrows\t%d\n" % grid.nrows)
fp.write("xllcorner\t%f\n" % grid.x0_corner)
if grid.sizey < 0:
fp.write("yllcorner\t%f\n" % (grid.y0_corner + grid.sizey * grid.nrows))
else:
fp.write("yllcorner\t%f\n" % grid.y0_corner)
assert np.abs(grid.sizex) == np.abs(grid.sizey)
fp.write("cellsize\t%f\n" % grid.sizex)
fp.write("NODATA_value\t%f\n" % nodataval)
DelimitedSpatialGrid.write(grid, fp, ' ', nodataval)
| {'content_hash': 'b9d9df31376ca427f52fd012ca08c2fa', 'timestamp': '', 'source': 'github', 'line_count': 136, 'max_line_length': 155, 'avg_line_length': 38.88970588235294, 'alnum_prop': 0.5685384760824352, 'repo_name': 'jrising/research-common', 'id': '081ff1789b906546617de1fb539fa0d5be29b2ca', 'size': '5289', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'python/geogrid/spacegrid.py', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'HTML', 'bytes': '29716'}, {'name': 'Python', 'bytes': '77850'}, {'name': 'R', 'bytes': '53879'}, {'name': 'Shell', 'bytes': '1521'}]} |
using System;
using Microsoft.Framework.Runtime;
namespace DefaultSiteTemplate
{
[AssemblyNeutral]
public interface IDocUrlBuilder
{
string Url(string docId);
}
} | {'content_hash': '10dd6455fd07a93b47f32c35bd715e90', 'timestamp': '', 'source': 'github', 'line_count': 11, 'max_line_length': 35, 'avg_line_length': 16.818181818181817, 'alnum_prop': 0.7189189189189189, 'repo_name': 'aspnetvnexthackathon/kdoc', 'id': '8675f5f6abb11c0973e8c3d66b0674ecc1b3eece', 'size': '187', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/DefaultSiteTemplate/IDocUrlBuilder.cs', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'C#', 'bytes': '115277'}, {'name': 'CSS', 'bytes': '124288'}, {'name': 'Shell', 'bytes': '1379'}]} |
#ifndef CHANNELSECTION_H_INC
#define CHANNELSECTION_H_INC
#include <json/json.h>
#include "configsection.h"
class ChannelSection : public ConfigSection
{
private:
static const int DefaultTopicLen=390;
unsigned int topiclen;
public:
void set_defaults();
void process(const Json::Value);
void verify() const;
inline unsigned int get_topiclen() { return topiclen; }
};
#endif
| {'content_hash': 'b7f5b65e53b2d79cb59ad016d5aee2c7', 'timestamp': '', 'source': 'github', 'line_count': 22, 'max_line_length': 57, 'avg_line_length': 17.772727272727273, 'alnum_prop': 0.7391304347826086, 'repo_name': 'oftc/oftc-ircd', 'id': '80e20ce19a43321682db44e0fdc066fc5f287f81', 'size': '1490', 'binary': False, 'copies': '1', 'ref': 'refs/heads/develop', 'path': 'include/channelsection.h', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'C', 'bytes': '12555'}, {'name': 'C++', 'bytes': '229190'}, {'name': 'Python', 'bytes': '27728'}, {'name': 'Shell', 'bytes': '183'}]} |
package simpleci.worker.queue;
import com.rabbitmq.client.Channel;
import com.rabbitmq.client.Connection;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import simpleci.shared.worker.options.GceWorkerOptions;
import simpleci.shared.worker.options.LocalWorkerOptions;
import simpleci.worker.AppParameters;
import simpleci.worker.state.WorkerState;
import simpleci.worker.job.JobProcessor;
import java.io.IOException;
import java.util.Date;
public class JobConsumer implements Runnable {
private final static Logger logger = LoggerFactory.getLogger(JobConsumer.class);
private final Connection connection;
private final JobProcessor processor;
private final AppParameters parameters;
private final WorkerState state;
public JobConsumer(
Connection connection,
JobProcessor jobProcessor,
AppParameters parameters, WorkerState state) {
this.connection = connection;
this.processor = jobProcessor;
this.parameters = parameters;
this.state = state;
}
@Override
public void run() {
try {
String queueName = queueName(parameters);
Channel channel = connection.createChannel();
channel.queueDeclare(queueName, true, false, false, null);
channel.basicQos(1);
BlockingConsumer consumer = new BlockingConsumer(channel);
channel.basicConsume(queueName, false, consumer);
logger.info("Listen on queue {}", queueName);
while (true) {
try {
BlockingConsumer.Delivery delivery = getDelivery(consumer);
if (delivery == null) {
logger.info("Consumer receive timeout reached. Worker will be exit now");
return;
}
String message = new String(delivery.getBody());
processor.process(message);
channel.basicAck(delivery.getEnvelope().getDeliveryTag(), false);
} catch (InterruptedException | IOException e) {
logger.error("", e);
return;
}
}
} catch (IOException e) {
logger.error("Error while consuming job messages", e);
return;
}
}
private String queueName(AppParameters parameters) {
if(parameters.workerOptions instanceof LocalWorkerOptions) {
return "local";
}else if(parameters.workerOptions instanceof GceWorkerOptions) {
return String.format("gcp.%d", ((GceWorkerOptions) parameters.workerOptions).gceProviderId);
} else {
logger.error(String.format("Unknown provider: %s", parameters.workerOptions.getClass().getName()));
return "";
}
}
private BlockingConsumer.Delivery getDelivery(BlockingConsumer consumer) throws InterruptedException {
if (parameters.exitIfInactive) {
int secondsDiff = seconds(state.startedAt, new Date());
int waitTime;
if (secondsDiff <= parameters.minimumRunningTime) {
waitTime = parameters.minimumRunningTime - secondsDiff;
} else {
int granularityCount = (int) Math.ceil((double) secondsDiff / parameters.timeGranulatity);
waitTime = parameters.timeGranulatity * granularityCount - secondsDiff;
}
logger.info(String.format("Waiting for message %d seconds", waitTime));
return consumer.nextDelivery(waitTime);
} else {
return consumer.nextDelivery();
}
}
private int seconds(Date start, Date end) {
return (int) ((end.getTime() - start.getTime()) / 1000);
}
}
| {'content_hash': '64bf8de60cd3a4c14d37bb19c864babd', 'timestamp': '', 'source': 'github', 'line_count': 101, 'max_line_length': 111, 'avg_line_length': 37.59405940594059, 'alnum_prop': 0.6228601527521728, 'repo_name': 'simpleci/simpleci', 'id': '71200e1252f62e04cb85f6d3e6934528fb2764a8', 'size': '3797', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/backend/worker/src/main/java/simpleci/worker/queue/JobConsumer.java', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '69187'}, {'name': 'HTML', 'bytes': '75915'}, {'name': 'Java', 'bytes': '186815'}, {'name': 'JavaScript', 'bytes': '39839'}, {'name': 'PHP', 'bytes': '147524'}, {'name': 'Shell', 'bytes': '10368'}]} |
from pyalgotrade.bitstamp import barfeed
from pyalgotrade.bitstamp import broker
from pyalgotrade import strategy
from pyalgotrade.technical import ma
from pyalgotrade.technical import cross
class Strategy(strategy.BaseStrategy):
def __init__(self, feed, brk):
strategy.BaseStrategy.__init__(self, feed, brk)
smaPeriod = 20
self.__instrument = "BTC"
self.__prices = feed[self.__instrument].getCloseDataSeries()
self.__sma = ma.SMA(self.__prices, smaPeriod)
self.__bid = None
self.__ask = None
self.__position = None
self.__posSize = 0.05
# Subscribe to order book update events to get bid/ask prices to trade.
feed.getOrderBookUpdateEvent().subscribe(self.__onOrderBookUpdate)
def __onOrderBookUpdate(self, orderBookUpdate):
bid = orderBookUpdate.getBidPrices()[0]
ask = orderBookUpdate.getAskPrices()[0]
if bid != self.__bid or ask != self.__ask:
self.__bid = bid
self.__ask = ask
self.info("Order book updated. Best bid: %s. Best ask: %s" % (self.__bid, self.__ask))
def onEnterOk(self, position):
self.info("Position opened at %s" % (position.getEntryOrder().getExecutionInfo().getPrice()))
def onEnterCanceled(self, position):
self.info("Position entry canceled")
self.__position = None
def onExitOk(self, position):
self.__position = None
self.info("Position closed at %s" % (position.getExitOrder().getExecutionInfo().getPrice()))
def onExitCanceled(self, position):
# If the exit was canceled, re-submit it.
self.__position.exitLimit(self.__bid)
def onBars(self, bars):
bar = bars[self.__instrument]
self.info("Price: %s. Volume: %s." % (bar.getClose(), bar.getVolume()))
# Wait until we get the current bid/ask prices.
if self.__ask is None:
return
# If a position was not opened, check if we should enter a long position.
if self.__position is None:
if cross.cross_above(self.__prices, self.__sma) > 0:
self.info("Entry signal. Buy at %s" % (self.__ask))
self.__position = self.enterLongLimit(self.__instrument, self.__ask, self.__posSize, True)
# Check if we have to close the position.
elif not self.__position.exitActive() and cross.cross_below(self.__prices, self.__sma) > 0:
self.info("Exit signal. Sell at %s" % (self.__bid))
self.__position.exitLimit(self.__bid)
def main():
barFeed = barfeed.LiveTradeFeed()
brk = broker.PaperTradingBroker(1000, barFeed)
strat = Strategy(barFeed, brk)
strat.run()
if __name__ == "__main__":
main()
| {'content_hash': '6b0e6799f3b70428df3bb75c427f6fad', 'timestamp': '', 'source': 'github', 'line_count': 74, 'max_line_length': 106, 'avg_line_length': 37.310810810810814, 'alnum_prop': 0.6182542557044549, 'repo_name': 'iminrhythm/iirmerl', 'id': 'f0f57508334a4fc712a10a5afa7f90a4290d263b', 'size': '2871', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'p2btstmp.py', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Python', 'bytes': '17540'}]} |
//
// FWTCustomPropertyMapping.h
// FWTMappingKit
//
// Created by Jonathan Flintham on 22/08/2014.
//
//
/**
An instance of `FWTCustomPropertyMapping` specifies a custom mapping from a single `sourceKeyPath` on a source object to a single `destinationKey` on a destination object. Additionally, it is possible to specify a custom `relationshipMappingKey`, which will subsequently be passed to a related NSManagedObject subclass in order that it can provide a differentiated mapping for varying relationship contexts.
Arrays of `FWTCustomPropertyMapping` objects are intended to be returned by overridden implmentations of `fwt_customPropertyMappingsForMappingKey:` in `NSManagedObject+FWTRestKitMapping`.
*/
@interface FWTCustomPropertyMapping : NSObject
/// @name Properties
/**
The keyPath from which the source value will be accessed.
*/
@property (nonatomic, readonly) NSString *sourceKeyPath;
/**
The keyPath from which the transformed value will be set.
*/
@property (nonatomic, readonly) NSString *destinationKey;
/**
An optional mappingKey which will determine the mapping configuration of objects mapped to the relationship specified by `destinationKey`.
*/
@property (nonatomic, readonly) NSString *relationshipMappingKey;
/// @name Initialisation
/**
Allows custom configuration of a property mapping.
@param sourceKeyPath The keyPath on the source object from which a value will be retrieved, transformed, and then mapped to the destination object.
@param destinationKey The key on the destination object to which the transformed value from the source object will be mapped. Optionally provide `nil` or empty to explicitely ignore `sourceKeyPath` during the mapping process, i.e. if you do not want the property to be mapped automatically via entity reflection.
@return The newly initialised `FWTCustomPropertyMapping` object.
*/
- (instancetype)initWithSourceKeyPath:(NSString *)sourceKeyPath mappedToDestinationKey:(NSString *)destinationKey;
/**
Like `initWithSourceKeyPath:mappedToDestinationKey:`, but optionally allows for relationship mappings to be assigned to alternative mappings differentiated with a mappingKey. For example, a Person entity might need a different mapping configuration when it refers to an employer than to an employee.
@param sourceKeyPath The keyPath on the source object from which a value will be retrieved, transformed, and then mapped to the destination object.
@param destinationKey The key on the destination object to which the transformed value from the source object will be mapped. Optionally provide `nil` or empty to explicitely ignore the `sourceKeyPath` during the mapping process, i.e. if you do not want the property to be mapped automatically via entity reflection.
@param relationshipMappingKey The mappingKey that differentiate the mapping configuration for the relationship.
@return The newly initialised `FWTCustomPropertyMapping` object.
@warning `destinationKey` should refer to a relationship on the destination object
*/
- (instancetype)initWithSourceKeyPath:(NSString *)sourceKeyPath mappedToDestinationKey:(NSString *)destinationKey withRelationshipMappingKey:(NSString *)relationshipMappingKey;
/**
Convenience for creating a batch of simple FWTCustomPropertyMapping instances. `initWithSourceKeyPath:mappedToDestinationKey:` is called for each `sourceKeyPath`:`destinationKey` pair provided in `dictionary`, and the array of resulting configurations is returned.
When it is necessary to specify a custom mappingKey for objects mapped to a relationship, use `initWithSourceKeyPath:mappedToDestinationKey:relationshipMappingKey:` instead.
@param dictionary Dictionary where keys are `sourceKeyPath`s and values are corresponding `destinationKey`s.
@return An array of instances of FWTMappingConfiguraton.
*/
+ (NSArray *)mappingConfigurationsFromDictionary:(NSDictionary *)dictionary;
@end
| {'content_hash': '99e41cd4222d67271448f4ac410269c6', 'timestamp': '', 'source': 'github', 'line_count': 70, 'max_line_length': 408, 'avg_line_length': 55.97142857142857, 'alnum_prop': 0.8083205717202654, 'repo_name': 'FutureWorkshops/FWTMappingKit', 'id': '086b87b8184af48ce888f4adebaab4cb90007bd7', 'size': '3918', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'FWTMappingKit/FWTCustomPropertyMapping.h', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Objective-C', 'bytes': '44417'}, {'name': 'Ruby', 'bytes': '1262'}]} |
"""main.py
HTTP controller handlers for memcache & task queue access
Jasen Carroll
Sept 15, 2015
"""
import webapp2
from google.appengine.api import app_identity
from google.appengine.api import mail
from conference import ConferenceApi
class SetAnnouncementHandler(webapp2.RequestHandler):
def get(self):
"""Set Announcement in Memcache."""
ConferenceApi._cacheAnnouncement()
self.response.set_status(204)
class SendConfirmationEmailHandler(webapp2.RequestHandler):
def post(self):
"""Send email confirming Conference creation."""
mail.send_mail(
'noreply@%s.appspotmail.com' % (
app_identity.get_application_id()), # from
self.request.get('email'), # to
'You created a new Conference!', # subj
'Hi, you have created a following ' # body
'conference:\r\n\r\n%s' % self.request.get(
'conferenceInfo')
)
class SetFeaturedSpeakerHandler(webapp2.RequestHandler):
def post(self):
"""Set Featured Speaker in Memcahce."""
ConferenceApi._cacheSpeaker(self.request.get('speaker'))
self.response.set_status(204)
app = webapp2.WSGIApplication([
('/crons/set_announcement', SetAnnouncementHandler),
('/tasks/send_confirmation_email', SendConfirmationEmailHandler),
('/tasks/set_featured_speaker', SetFeaturedSpeakerHandler),
], debug=True)
| {'content_hash': '5e26ad7a5ad8363e6a20467f46e1d179', 'timestamp': '', 'source': 'github', 'line_count': 46, 'max_line_length': 69, 'avg_line_length': 31.891304347826086, 'alnum_prop': 0.6523517382413088, 'repo_name': 'jasenc/conference', 'id': '8a51168cb1335ad5c09a3c3b8d2b273a81165cee', 'size': '1490', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'main.py', 'mode': '33261', 'license': 'apache-2.0', 'language': [{'name': 'HTML', 'bytes': '23913'}, {'name': 'JavaScript', 'bytes': '32837'}, {'name': 'Python', 'bytes': '41177'}]} |
var _ = require('lodash');
var UsersManager = require("../lib/UsersManager");
var DatabaseManager = require("../lib/DatabaseManager");
var Utils = require("../lib/Utils");
var Const = require("../const");
var SocketHandlerBase = require("./SocketHandlerBase");
var SocketAPIHandler = require('../SocketAPI/SocketAPIHandler');
var SendMessageActionHandler = function(){
}
var SendMessageLogic = require('../Logics/SendMessage');
var BridgeManager = require('../lib/BridgeManager');
_.extend(SendMessageActionHandler.prototype,SocketHandlerBase.prototype);
SendMessageActionHandler.prototype.attach = function(io,socket){
var self = this;
/**
* @api {socket} "sendMessage" Send New Message
* @apiName Send Message
* @apiGroup Socket
* @apiDescription Send new message by socket
* @apiParam {string} roomID Room ID
* @apiParam {string} userID User ID
* @apiParam {string} type Message Type. 1:Text 2:File 3:Location
* @apiParam {string} message Message if type == 1
* @apiParam {string} fileID File ID if type == 2
* @apiParam {object} location lat and lng if type == 3
*
*/
socket.on('sendMessage', function(param){
if(Utils.isEmpty(param.roomID)){
socket.emit('socketerror', {code:Const.resCodeSocketSendMessageNoRoomID});
return;
}
if(Utils.isEmpty(param.userID)){
socket.emit('socketerror', {code:Const.resCodeSocketSendMessageNoUserId});
return;
}
if(Utils.isEmpty(param.type)){
socket.emit('socketerror', {code:Const.resCodeSocketSendMessageNoType});
return;
}
if(param.type == Const.messageTypeText && Utils.isEmpty(param.message)){
socket.emit('socketerror', {code:Const.resCodeSocketSendMessageNoMessage});
return;
}
if(param.type == Const.messageTypeLocation && (
Utils.isEmpty(param.location) ||
Utils.isEmpty(param.location.lat) ||
Utils.isEmpty(param.location.lng))){
socket.emit('socketerror', {code:Const.resCodeSocketSendMessageNoLocation});
return;
}
var userID = param.userID;
BridgeManager.hook('sendMessage',param,function(result){
if(result == null || result.canSend){
var userID = param.userID;
SendMessageLogic.execute(userID,param,function(result){
},function(err,code){
if(err){
socket.emit('socketerror', {code:Const.resCodeSocketSendMessageFail});
}else{
socket.emit('socketerror', {code:code});
}
});
}
});
});
}
module["exports"] = new SendMessageActionHandler(); | {'content_hash': 'ecce57b86985671b3872b80cf9f8d82a', 'timestamp': '', 'source': 'github', 'line_count': 106, 'max_line_length': 103, 'avg_line_length': 31.38679245283019, 'alnum_prop': 0.5163811241358581, 'repo_name': 'cloverstudio/Spika', 'id': 'e0df70dc496b93b890af7ec48a8557a71ffd1ced', 'size': '3328', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'web/src/server/SocketAPI/SendMessageActionHandler.js', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '16489'}, {'name': 'HTML', 'bytes': '23144'}, {'name': 'Java', 'bytes': '457558'}, {'name': 'JavaScript', 'bytes': '227203'}, {'name': 'Objective-C', 'bytes': '282645'}, {'name': 'Ruby', 'bytes': '323'}]} |
#include <linux/kernel.h>
#include <linux/slab.h>
#include <linux/firmware.h>
#include <linux/device.h>
#include <linux/module.h>
#include "hermes.h"
#include "hermes_dld.h"
#include "orinoco.h"
#include "fw.h"
/* End markers (for Symbol firmware only) */
#define TEXT_END 0x1A /* End of text header */
struct fw_info {
char *pri_fw;
char *sta_fw;
char *ap_fw;
u32 pda_addr;
u16 pda_size;
};
static const struct fw_info orinoco_fw[] = {
{ NULL, "agere_sta_fw.bin", "agere_ap_fw.bin", 0x00390000, 1000 },
{ NULL, "prism_sta_fw.bin", "prism_ap_fw.bin", 0, 1024 },
{ "symbol_sp24t_prim_fw", "symbol_sp24t_sec_fw", NULL, 0x00003100, 512 }
};
MODULE_FIRMWARE("agere_sta_fw.bin");
MODULE_FIRMWARE("agere_ap_fw.bin");
MODULE_FIRMWARE("prism_sta_fw.bin");
MODULE_FIRMWARE("prism_ap_fw.bin");
MODULE_FIRMWARE("symbol_sp24t_prim_fw");
MODULE_FIRMWARE("symbol_sp24t_sec_fw");
/* Structure used to access fields in FW
* Make sure LE decoding macros are used
*/
struct orinoco_fw_header {
char hdr_vers[6]; /* ASCII string for header version */
__le16 headersize; /* Total length of header */
__le32 entry_point; /* NIC entry point */
__le32 blocks; /* Number of blocks to program */
__le32 block_offset; /* Offset of block data from eof header */
__le32 pdr_offset; /* Offset to PDR data from eof header */
__le32 pri_offset; /* Offset to primary plug data */
__le32 compat_offset; /* Offset to compatibility data*/
char signature[0]; /* FW signature length headersize-20 */
} __packed;
/* Check the range of various header entries. Return a pointer to a
* description of the problem, or NULL if everything checks out. */
static const char *validate_fw(const struct orinoco_fw_header *hdr, size_t len)
{
u16 hdrsize;
if (len < sizeof(*hdr))
return "image too small";
if (memcmp(hdr->hdr_vers, "HFW", 3) != 0)
return "format not recognised";
hdrsize = le16_to_cpu(hdr->headersize);
if (hdrsize > len)
return "bad headersize";
if ((hdrsize + le32_to_cpu(hdr->block_offset)) > len)
return "bad block offset";
if ((hdrsize + le32_to_cpu(hdr->pdr_offset)) > len)
return "bad PDR offset";
if ((hdrsize + le32_to_cpu(hdr->pri_offset)) > len)
return "bad PRI offset";
if ((hdrsize + le32_to_cpu(hdr->compat_offset)) > len)
return "bad compat offset";
/* TODO: consider adding a checksum or CRC to the firmware format */
return NULL;
}
#if defined(CONFIG_HERMES_CACHE_FW_ON_INIT) || defined(CONFIG_PM_SLEEP)
static inline const struct firmware *
orinoco_cached_fw_get(struct orinoco_private *priv, bool primary)
{
if (primary)
return priv->cached_pri_fw;
else
return priv->cached_fw;
}
#else
#define orinoco_cached_fw_get(priv, primary) (NULL)
#endif
/* Download either STA or AP firmware into the card. */
static int
orinoco_dl_firmware(struct orinoco_private *priv,
const struct fw_info *fw,
int ap)
{
/* Plug Data Area (PDA) */
__le16 *pda;
struct hermes *hw = &priv->hw;
const struct firmware *fw_entry;
const struct orinoco_fw_header *hdr;
const unsigned char *first_block;
const void *end;
const char *firmware;
const char *fw_err;
struct device *dev = priv->dev;
int err = 0;
pda = kzalloc(fw->pda_size, GFP_KERNEL);
if (!pda)
return -ENOMEM;
if (ap)
firmware = fw->ap_fw;
else
firmware = fw->sta_fw;
dev_dbg(dev, "Attempting to download firmware %s\n", firmware);
/* Read current plug data */
err = hw->ops->read_pda_h(hw, pda, fw->pda_addr, fw->pda_size);
dev_dbg(dev, "Read PDA returned %d\n", err);
if (err)
goto free;
if (!orinoco_cached_fw_get(priv, false)) {
err = request_firmware(&fw_entry, firmware, priv->dev);
if (err) {
dev_err(dev, "Cannot find firmware %s\n", firmware);
err = -ENOENT;
goto free;
}
} else
fw_entry = orinoco_cached_fw_get(priv, false);
hdr = (const struct orinoco_fw_header *) fw_entry->data;
fw_err = validate_fw(hdr, fw_entry->size);
if (fw_err) {
dev_warn(dev, "Invalid firmware image detected (%s). "
"Aborting download\n", fw_err);
err = -EINVAL;
goto abort;
}
/* Enable aux port to allow programming */
err = hw->ops->program_init(hw, le32_to_cpu(hdr->entry_point));
dev_dbg(dev, "Program init returned %d\n", err);
if (err != 0)
goto abort;
/* Program data */
first_block = (fw_entry->data +
le16_to_cpu(hdr->headersize) +
le32_to_cpu(hdr->block_offset));
end = fw_entry->data + fw_entry->size;
err = hermes_program(hw, first_block, end);
dev_dbg(dev, "Program returned %d\n", err);
if (err != 0)
goto abort;
/* Update production data */
first_block = (fw_entry->data +
le16_to_cpu(hdr->headersize) +
le32_to_cpu(hdr->pdr_offset));
err = hermes_apply_pda_with_defaults(hw, first_block, end, pda,
&pda[fw->pda_size / sizeof(*pda)]);
dev_dbg(dev, "Apply PDA returned %d\n", err);
if (err)
goto abort;
/* Tell card we've finished */
err = hw->ops->program_end(hw);
dev_dbg(dev, "Program end returned %d\n", err);
if (err != 0)
goto abort;
/* Check if we're running */
dev_dbg(dev, "hermes_present returned %d\n", hermes_present(hw));
abort:
/* If we requested the firmware, release it. */
if (!orinoco_cached_fw_get(priv, false))
release_firmware(fw_entry);
free:
kfree(pda);
return err;
}
/*
* Process a firmware image - stop the card, load the firmware, reset
* the card and make sure it responds. For the secondary firmware take
* care of the PDA - read it and then write it on top of the firmware.
*/
static int
symbol_dl_image(struct orinoco_private *priv, const struct fw_info *fw,
const unsigned char *image, const void *end,
int secondary)
{
struct hermes *hw = &priv->hw;
int ret = 0;
const unsigned char *ptr;
const unsigned char *first_block;
/* Plug Data Area (PDA) */
__le16 *pda = NULL;
/* Binary block begins after the 0x1A marker */
ptr = image;
while (*ptr++ != TEXT_END);
first_block = ptr;
/* Read the PDA from EEPROM */
if (secondary) {
pda = kzalloc(fw->pda_size, GFP_KERNEL);
if (!pda)
return -ENOMEM;
ret = hw->ops->read_pda_h(hw, pda, fw->pda_addr, fw->pda_size);
if (ret)
goto free;
}
/* Stop the firmware, so that it can be safely rewritten */
if (priv->stop_fw) {
ret = priv->stop_fw(priv, 1);
if (ret)
goto free;
}
/* Program the adapter with new firmware */
ret = hermes_program(hw, first_block, end);
if (ret)
goto free;
/* Write the PDA to the adapter */
if (secondary) {
size_t len = hermes_blocks_length(first_block, end);
ptr = first_block + len;
ret = hermes_apply_pda(hw, ptr, end, pda,
&pda[fw->pda_size / sizeof(*pda)]);
kfree(pda);
if (ret)
return ret;
}
/* Run the firmware */
if (priv->stop_fw) {
ret = priv->stop_fw(priv, 0);
if (ret)
return ret;
}
/* Reset hermes chip and make sure it responds */
ret = hw->ops->init(hw);
/* hermes_reset() should return 0 with the secondary firmware */
if (secondary && ret != 0)
return -ENODEV;
/* And this should work with any firmware */
if (!hermes_present(hw))
return -ENODEV;
return 0;
free:
kfree(pda);
return ret;
}
/*
* Download the firmware into the card, this also does a PCMCIA soft
* reset on the card, to make sure it's in a sane state.
*/
static int
symbol_dl_firmware(struct orinoco_private *priv,
const struct fw_info *fw)
{
struct device *dev = priv->dev;
int ret;
const struct firmware *fw_entry;
if (!orinoco_cached_fw_get(priv, true)) {
if (request_firmware(&fw_entry, fw->pri_fw, priv->dev) != 0) {
dev_err(dev, "Cannot find firmware: %s\n", fw->pri_fw);
return -ENOENT;
}
} else
fw_entry = orinoco_cached_fw_get(priv, true);
/* Load primary firmware */
ret = symbol_dl_image(priv, fw, fw_entry->data,
fw_entry->data + fw_entry->size, 0);
if (!orinoco_cached_fw_get(priv, true))
release_firmware(fw_entry);
if (ret) {
dev_err(dev, "Primary firmware download failed\n");
return ret;
}
if (!orinoco_cached_fw_get(priv, false)) {
if (request_firmware(&fw_entry, fw->sta_fw, priv->dev) != 0) {
dev_err(dev, "Cannot find firmware: %s\n", fw->sta_fw);
return -ENOENT;
}
} else
fw_entry = orinoco_cached_fw_get(priv, false);
/* Load secondary firmware */
ret = symbol_dl_image(priv, fw, fw_entry->data,
fw_entry->data + fw_entry->size, 1);
if (!orinoco_cached_fw_get(priv, false))
release_firmware(fw_entry);
if (ret)
dev_err(dev, "Secondary firmware download failed\n");
return ret;
}
int orinoco_download(struct orinoco_private *priv)
{
int err = 0;
/* Reload firmware */
switch (priv->firmware_type) {
case FIRMWARE_TYPE_AGERE:
/* case FIRMWARE_TYPE_INTERSIL: */
err = orinoco_dl_firmware(priv,
&orinoco_fw[priv->firmware_type], 0);
break;
case FIRMWARE_TYPE_SYMBOL:
err = symbol_dl_firmware(priv,
&orinoco_fw[priv->firmware_type]);
break;
case FIRMWARE_TYPE_INTERSIL:
break;
}
/* TODO: if we fail we probably need to reinitialise
* the driver */
return err;
}
#if defined(CONFIG_HERMES_CACHE_FW_ON_INIT) || defined(CONFIG_PM_SLEEP)
void orinoco_cache_fw(struct orinoco_private *priv, int ap)
{
const struct firmware *fw_entry = NULL;
const char *pri_fw;
const char *fw;
pri_fw = orinoco_fw[priv->firmware_type].pri_fw;
if (ap)
fw = orinoco_fw[priv->firmware_type].ap_fw;
else
fw = orinoco_fw[priv->firmware_type].sta_fw;
if (pri_fw) {
if (request_firmware(&fw_entry, pri_fw, priv->dev) == 0)
priv->cached_pri_fw = fw_entry;
}
if (fw) {
if (request_firmware(&fw_entry, fw, priv->dev) == 0)
priv->cached_fw = fw_entry;
}
}
void orinoco_uncache_fw(struct orinoco_private *priv)
{
if (priv->cached_pri_fw)
release_firmware(priv->cached_pri_fw);
if (priv->cached_fw)
release_firmware(priv->cached_fw);
priv->cached_pri_fw = NULL;
priv->cached_fw = NULL;
}
#endif
| {'content_hash': '71687f7ef5d63d0da0adea5f67374916', 'timestamp': '', 'source': 'github', 'line_count': 387, 'max_line_length': 79, 'avg_line_length': 25.41343669250646, 'alnum_prop': 0.6523640061006609, 'repo_name': 'Xperia-Nicki/android_platform_sony_nicki', 'id': 'a2434f8d6711984e5bcb158e2879d22c95915182', 'size': '9921', 'binary': False, 'copies': '58', 'ref': 'refs/heads/master', 'path': 'external/compat-wireless/drivers/net/wireless/orinoco/fw.c', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Ada', 'bytes': '89080'}, {'name': 'Assembly', 'bytes': '212775'}, {'name': 'Awk', 'bytes': '19252'}, {'name': 'C', 'bytes': '68667466'}, {'name': 'C#', 'bytes': '55625'}, {'name': 'C++', 'bytes': '54670920'}, {'name': 'CLIPS', 'bytes': '12224'}, {'name': 'CSS', 'bytes': '283405'}, {'name': 'D', 'bytes': '1931'}, {'name': 'Java', 'bytes': '4882'}, {'name': 'JavaScript', 'bytes': '19597804'}, {'name': 'Objective-C', 'bytes': '5849156'}, {'name': 'PHP', 'bytes': '17224'}, {'name': 'Pascal', 'bytes': '42411'}, {'name': 'Perl', 'bytes': '1632149'}, {'name': 'Prolog', 'bytes': '214621'}, {'name': 'Python', 'bytes': '3493321'}, {'name': 'R', 'bytes': '290'}, {'name': 'Ruby', 'bytes': '78743'}, {'name': 'Scilab', 'bytes': '554'}, {'name': 'Shell', 'bytes': '265637'}, {'name': 'TypeScript', 'bytes': '45459'}, {'name': 'XSLT', 'bytes': '11219'}]} |
package ro.nextreports.designer.property;
import com.l2fprod.common.beans.editor.AbstractPropertyEditor;
import com.l2fprod.common.swing.renderer.DefaultCellRenderer;
import com.l2fprod.common.swing.PercentLayout;
import com.l2fprod.common.swing.ComponentFactory;
import ro.nextreports.engine.condition.FormattingConditions;
import javax.swing.*;
import ro.nextreports.designer.util.I18NSupport;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
import java.util.List;
/**
* User: mihai.panaitescu
* Date: 23-Apr-2010
* Time: 11:57:00
*/
public class FormattingConditionsPropertyEditor extends AbstractPropertyEditor {
private DefaultCellRenderer label;
private JButton button;
private FormattingConditions conditions;
private String type;
private String bandName;
public FormattingConditionsPropertyEditor(String type, String bandName) {
this.type = type;
this.bandName = bandName;
JPanel conditionEditor = new JPanel(new PercentLayout(PercentLayout.HORIZONTAL, 0));
editor = conditionEditor;
conditionEditor.add("*", label = new DefaultCellRenderer());
label.setOpaque(false);
conditionEditor.add(button = ComponentFactory.Helper.getFactory().createMiniButton());
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
selectConditions();
}
});
conditionEditor.add(button = ComponentFactory.Helper.getFactory().createMiniButton());
button.setText("X");
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
selectNull();
}
});
conditionEditor.setOpaque(false);
}
public Object getValue() {
return conditions;
}
public void setValue(Object value) {
conditions = (FormattingConditions) value;
label.setValue(value);
}
public void setType(String type) {
this.type = type;
}
protected void selectConditions() {
FormattingConditions selected = FormattingConditionsChooser.showDialog(editor, I18NSupport.getString("condition.dialog.title"), conditions, type, bandName);
if (selected != null) {
FormattingConditions oldC = conditions;
FormattingConditions newC = selected;
label.setValue(newC);
conditions = newC;
firePropertyChange(oldC, newC);
}
}
protected void selectNull() {
Object oldPattern = conditions;
label.setValue(null);
conditions = null;
firePropertyChange(oldPattern, null);
}
}
| {'content_hash': '153508913d4d0f87e101ff5248d9d03c', 'timestamp': '', 'source': 'github', 'line_count': 87, 'max_line_length': 164, 'avg_line_length': 31.25287356321839, 'alnum_prop': 0.6792938580360427, 'repo_name': 'nextreports/nextreports-designer', 'id': 'e8e7f8b45d4e2e9208c63855fe748e8245812bf2', 'size': '3523', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/ro/nextreports/designer/property/FormattingConditionsPropertyEditor.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Batchfile', 'bytes': '80'}, {'name': 'Java', 'bytes': '3392568'}, {'name': 'Shell', 'bytes': '373'}]} |
@interface RHPacketFrame : NSObject <RHSocketPacket>
@end
| {'content_hash': '07096440f5f78daaafc8e3162c3508bf', 'timestamp': '', 'source': 'github', 'line_count': 3, 'max_line_length': 52, 'avg_line_length': 19.666666666666668, 'alnum_prop': 0.7966101694915254, 'repo_name': 'edison9888/RHSocketKit', 'id': 'c75ac0e1b540f4a53e065fcf02d5a79d12eb36d2', 'size': '262', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'RHSocketKit/Core/RHPacketFrame.h', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'C', 'bytes': '401'}, {'name': 'Objective-C', 'bytes': '32728'}, {'name': 'Ruby', 'bytes': '1385'}]} |
package com.lmn.Arbiter_Android.DatabaseHelpers.Migrations;
import com.lmn.Arbiter_Android.DatabaseHelpers.TableHelpers.ServersHelper;
import android.database.sqlite.SQLiteDatabase;
public class UpgradeAppDbFrom2To3 implements Migration{
private String tempTableName;
public UpgradeAppDbFrom2To3(){
this.tempTableName = "temporaryServersTable";
}
public void migrate(SQLiteDatabase db){
createBackupTable(db);
createTableWithNewSchema(db);
moveDataOver(db);
dropTemporaryTable(db);
}
private void createBackupTable(SQLiteDatabase db){
String createBackupTable = "ALTER TABLE " + ServersHelper.SERVERS_TABLE_NAME + " RENAME TO " + tempTableName + ";";
db.execSQL(createBackupTable);
}
private void createTableWithNewSchema(SQLiteDatabase db){
ServersHelper.getServersHelper().createTable(db);
}
private void moveDataOver(SQLiteDatabase db){
String fields = ServersHelper._ID + "," +
ServersHelper.SERVER_NAME + "," +
ServersHelper.SERVER_URL + "," +
ServersHelper.SERVER_USERNAME + "," +
ServersHelper.SERVER_PASSWORD;
String moveDataOver = "INSERT INTO "+ ServersHelper.SERVERS_TABLE_NAME
+ " (" + fields + ") SELECT " + fields + " FROM " + tempTableName;
db.execSQL(moveDataOver);
}
private void dropTemporaryTable(SQLiteDatabase db){
db.execSQL("DROP TABLE " + tempTableName);
}
}
| {'content_hash': 'be0b90153c3e9d3cb35df61499bf7522', 'timestamp': '', 'source': 'github', 'line_count': 55, 'max_line_length': 117, 'avg_line_length': 25.21818181818182, 'alnum_prop': 0.7289113193943764, 'repo_name': 'ROGUE-JCTD/Arbiter-Android', 'id': '2d488c4a0146b2ff4ce621a6faa50195ceb63d0a', 'size': '1387', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'Arbiter-Android/src/com/lmn/Arbiter_Android/DatabaseHelpers/Migrations/UpgradeAppDbFrom2To3.java', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'C', 'bytes': '7006'}, {'name': 'CSS', 'bytes': '52499'}, {'name': 'HTML', 'bytes': '32077377'}, {'name': 'Java', 'bytes': '974332'}, {'name': 'JavaScript', 'bytes': '10097267'}, {'name': 'Python', 'bytes': '185976'}, {'name': 'Shell', 'bytes': '6299'}]} |
title: Amarok Easter Egg
author: Rami Taibah
permalink: amarok-easter-egg
tags: Amarok, Easter Egg, Linux
On [Answer.com](http://www.answers.com/topic/amarok-audio "Answer.com") Amarok page, it states that:
> Playing a song with the tag title as "Amarok" and the tag artist as "Mike Oldfield" produces the following [OSD](http://en.wikipedia.org/wiki/On-screen_display "OSD") popup:
>
> "One of [Mike Oldfield](http://en.wikipedia.org/wiki/Mike_Oldfield "Mike Oldfield")'s best pieces of work, [Amarok](http://en.wikipedia.org/wiki/Amarok_%28software%29 "Amarok"), inspired the name behind the audio-player you are currently using. Thanks for choosing **Amarok**!
>
> Mark Kretschmann
> Max Howell
> Chris Muehlhaeuser
> The many other people who have helped make Amarok what it is"
So I decided to test it out, here is a screen shot:

Oddly enough, Answer.com states that their source is [Wikipedia](http://www.wikipedia.com "Wikipedia"), but this easter egg does not appear in the [Amarok](http://en.wikipedia.org/wiki/Amarok_%28software%29 "Amarok") article of wiki.
| {'content_hash': '75b3d13f2271d9b5258ddbb2f76e9b56', 'timestamp': '', 'source': 'github', 'line_count': 23, 'max_line_length': 278, 'avg_line_length': 51.08695652173913, 'alnum_prop': 0.7480851063829788, 'repo_name': 'rtaibah/pelican-blog', 'id': '3a979967d5ed3d61c301b54b9065ee2f0ca98af5', 'size': '1175', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'content/blog/2007-12-27-amarok-easter-egg.markdown', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Makefile', 'bytes': '4329'}, {'name': 'Python', 'bytes': '5955'}, {'name': 'Shell', 'bytes': '2201'}]} |
package com.java110.community.listener.inspectionPoint;
import com.alibaba.fastjson.JSONArray;
import com.alibaba.fastjson.JSONObject;
import com.java110.community.dao.IInspectionServiceDao;
import com.java110.po.inspection.InspectionPointPo;
import com.java110.utils.constant.BusinessTypeConstant;
import com.java110.utils.constant.ResponseConstant;
import com.java110.utils.constant.StatusConstant;
import com.java110.utils.exception.ListenerExecuteException;
import com.java110.utils.util.Assert;
import com.java110.core.annotation.Java110Listener;
import com.java110.core.context.DataFlowContext;
import com.java110.entity.center.Business;
import org.slf4j.Logger;
import com.java110.core.log.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.transaction.annotation.Transactional;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* 删除巡检点信息 侦听
* <p>
* 处理节点
* 1、businessInspection:{} 巡检点基本信息节点
* 2、businessInspectionAttr:[{}] 巡检点属性信息节点
* 3、businessInspectionPhoto:[{}] 巡检点照片信息节点
* 4、businessInspectionCerdentials:[{}] 巡检点证件信息节点
* 协议地址 :https://github.com/java110/MicroCommunity/wiki/%E5%88%A0%E9%99%A4%E5%95%86%E6%88%B7%E4%BF%A1%E6%81%AF-%E5%8D%8F%E8%AE%AE
* Created by wuxw on 2018/5/18.
*/
@Java110Listener("deleteInspectionInfoListener")
@Transactional
public class DeleteInspectionInfoListener extends AbstractInspectionBusinessServiceDataFlowListener {
private final static Logger logger = LoggerFactory.getLogger(DeleteInspectionInfoListener.class);
@Autowired
IInspectionServiceDao inspectionServiceDaoImpl;
@Override
public int getOrder() {
return 3;
}
@Override
public String getBusinessTypeCd() {
return BusinessTypeConstant.BUSINESS_TYPE_DELETE_INSPECTION;
}
/**
* 根据删除信息 查出Instance表中数据 保存至business表 (状态写DEL) 方便撤单时直接更新回去
*
* @param dataFlowContext 数据对象
* @param business 当前业务对象
*/
@Override
protected void doSaveBusiness(DataFlowContext dataFlowContext, Business business) {
JSONObject data = business.getDatas();
Assert.notEmpty(data, "没有datas 节点,或没有子节点需要处理");
//处理 businessInspection 节点
if (data.containsKey(InspectionPointPo.class.getSimpleName())) {
Object _obj = data.get(InspectionPointPo.class.getSimpleName());
JSONArray businessInspections = null;
if (_obj instanceof JSONObject) {
businessInspections = new JSONArray();
businessInspections.add(_obj);
} else {
businessInspections = (JSONArray) _obj;
}
//JSONObject businessInspection = data.getJSONObject("businessInspection");
for (int _inspectionIndex = 0; _inspectionIndex < businessInspections.size(); _inspectionIndex++) {
JSONObject businessInspection = businessInspections.getJSONObject(_inspectionIndex);
doBusinessInspection(business, businessInspection);
if (_obj instanceof JSONObject) {
dataFlowContext.addParamOut("inspectionId", businessInspection.getString("inspectionId"));
}
}
}
}
/**
* 删除 instance数据
*
* @param dataFlowContext 数据对象
* @param business 当前业务对象
*/
@Override
protected void doBusinessToInstance(DataFlowContext dataFlowContext, Business business) {
String bId = business.getbId();
//Assert.hasLength(bId,"请求报文中没有包含 bId");
//巡检点信息
Map info = new HashMap();
info.put("bId", business.getbId());
info.put("operate", StatusConstant.OPERATE_DEL);
//巡检点信息
List<Map> businessInspectionInfos = inspectionServiceDaoImpl.getBusinessInspectionInfo(info);
if (businessInspectionInfos != null && businessInspectionInfos.size() > 0) {
for (int _inspectionIndex = 0; _inspectionIndex < businessInspectionInfos.size(); _inspectionIndex++) {
Map businessInspectionInfo = businessInspectionInfos.get(_inspectionIndex);
flushBusinessInspectionInfo(businessInspectionInfo, StatusConstant.STATUS_CD_INVALID);
inspectionServiceDaoImpl.updateInspectionInfoInstance(businessInspectionInfo);
dataFlowContext.addParamOut("inspectionId", businessInspectionInfo.get("inspection_id"));
}
}
}
/**
* 撤单
* 从business表中查询到DEL的数据 将instance中的数据更新回来
*
* @param dataFlowContext 数据对象
* @param business 当前业务对象
*/
@Override
protected void doRecover(DataFlowContext dataFlowContext, Business business) {
String bId = business.getbId();
//Assert.hasLength(bId,"请求报文中没有包含 bId");
Map info = new HashMap();
info.put("bId", bId);
info.put("statusCd", StatusConstant.STATUS_CD_INVALID);
Map delInfo = new HashMap();
delInfo.put("bId", business.getbId());
delInfo.put("operate", StatusConstant.OPERATE_DEL);
//巡检点信息
List<Map> inspectionInfo = inspectionServiceDaoImpl.getInspectionInfo(info);
if (inspectionInfo != null && inspectionInfo.size() > 0) {
//巡检点信息
List<Map> businessInspectionInfos = inspectionServiceDaoImpl.getBusinessInspectionInfo(delInfo);
//除非程序出错了,这里不会为空
if (businessInspectionInfos == null || businessInspectionInfos.size() == 0) {
throw new ListenerExecuteException(ResponseConstant.RESULT_CODE_INNER_ERROR, "撤单失败(inspection),程序内部异常,请检查! " + delInfo);
}
for (int _inspectionIndex = 0; _inspectionIndex < businessInspectionInfos.size(); _inspectionIndex++) {
Map businessInspectionInfo = businessInspectionInfos.get(_inspectionIndex);
flushBusinessInspectionInfo(businessInspectionInfo, StatusConstant.STATUS_CD_VALID);
inspectionServiceDaoImpl.updateInspectionInfoInstance(businessInspectionInfo);
}
}
}
/**
* 处理 businessInspection 节点
*
* @param business 总的数据节点
* @param businessInspection 巡检点节点
*/
private void doBusinessInspection(Business business, JSONObject businessInspection) {
Assert.jsonObjectHaveKey(businessInspection, "inspectionId", "businessInspection 节点下没有包含 inspectionId 节点");
if (businessInspection.getString("inspectionId").startsWith("-")) {
throw new ListenerExecuteException(ResponseConstant.RESULT_PARAM_ERROR, "inspectionId 错误,不能自动生成(必须已经存在的inspectionId)" + businessInspection);
}
//自动插入DEL
autoSaveDelBusinessInspection(business, businessInspection);
}
public IInspectionServiceDao getInspectionServiceDaoImpl() {
return inspectionServiceDaoImpl;
}
public void setInspectionServiceDaoImpl(IInspectionServiceDao inspectionServiceDaoImpl) {
this.inspectionServiceDaoImpl = inspectionServiceDaoImpl;
}
}
| {'content_hash': 'd5a3adc1752e9b3d6866cedab8108189', 'timestamp': '', 'source': 'github', 'line_count': 180, 'max_line_length': 152, 'avg_line_length': 39.03888888888889, 'alnum_prop': 0.6897680375693752, 'repo_name': 'java110/MicroCommunity', 'id': 'a1932829b7de057dc4b1144ae7164b1fa630fbc5', 'size': '7583', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'service-community/src/main/java/com/java110/community/listener/inspectionPoint/DeleteInspectionInfoListener.java', 'mode': '33261', 'license': 'apache-2.0', 'language': [{'name': 'Dockerfile', 'bytes': '737'}, {'name': 'HTML', 'bytes': '12981'}, {'name': 'Java', 'bytes': '19654092'}, {'name': 'JavaScript', 'bytes': '19296'}, {'name': 'Shell', 'bytes': '1121'}]} |
ACCEPTED
#### According to
International Plant Names Index
#### Published in
null
#### Original name
null
### Remarks
null | {'content_hash': '7aed0b32d3bb0ffcbeaff4837d8d51a9', 'timestamp': '', 'source': 'github', 'line_count': 13, 'max_line_length': 31, 'avg_line_length': 9.692307692307692, 'alnum_prop': 0.7063492063492064, 'repo_name': 'mdoering/backbone', 'id': 'ac90adcabd8e7ec1c442f6d62154110292783efb', 'size': '168', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'life/Plantae/Magnoliophyta/Magnoliopsida/Rosales/Rosaceae/Aria grantzii/README.md', 'mode': '33188', 'license': 'apache-2.0', 'language': []} |
<?xml version="1.0" encoding="utf-8"?>
<android.support.design.widget.CoordinatorLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent"
android:layout_height="match_parent" tools:context="com.marckregio.signature.SignatureActivity">
<android.support.design.widget.AppBarLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:theme="@style/AppTheme.AppBarOverlay">
<android.support.v7.widget.Toolbar
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
android:background="?attr/colorPrimary"
app:popupTheme="@style/AppTheme.PopupOverlay">
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingRight="10dp">
<TextView
android:id="@+id/title"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:text="Sign for "
android:textSize="20dp"
android:textStyle="bold"
android:textAllCaps="true"
android:gravity="center_vertical"
android:layout_alignParentLeft="true"/>
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_alignParentRight="true"
android:gravity="center">
<com.joanzapata.iconify.widget.IconTextView
android:id="@+id/delete"
style="@style/TextIconStyle"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:textSize="40dp"
android:text="{fa-trash-o}" />
<com.joanzapata.iconify.widget.IconTextView
android:id="@+id/done"
style="@style/TextIconStyle"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:textSize="40dp"
android:text="{fa-check-circle-o}"/>
</LinearLayout>
</RelativeLayout>
</android.support.v7.widget.Toolbar>
</android.support.design.widget.AppBarLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_marginTop="?attr/actionBarSize"
android:background="@color/white">
<com.simplify.ink.InkView
android:id="@+id/ink"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
</LinearLayout>
</android.support.design.widget.CoordinatorLayout>
| {'content_hash': '198925ec03dd06035d2da27abe33584d', 'timestamp': '', 'source': 'github', 'line_count': 78, 'max_line_length': 100, 'avg_line_length': 41.833333333333336, 'alnum_prop': 0.5488813974869752, 'repo_name': 'marckregio/maklib', 'id': 'cbe16179255811b44e1d5205745593dffb3d34ae', 'size': '3263', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'maklib-signature/src/main/res/layout/activity_signature.xml', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Java', 'bytes': '124778'}]} |
<?php
class Terminalor_DocParser_Template_Standard
extends Terminalor_DocParser_Template_Abstract
{
/**
* Description tag renderer
*
* @param string $commandName command name
* @param array $tags array of available tags
* @param string $tagName given tag name (description in this case)
* @return string rendered tag with style placeholders
*/
protected function _renderDescriptionTag($commandName, array $tags, $tagName)
{
$return = '';
$tag = Terminalor_Response_Styles::STYLE_TAG;
$isSingle = (1 >= count($this->getVars()));
if (!array_key_exists($tagName, $tags)) {
return $return;
}
$description = $tags[$tagName];
if ($isSingle) {
$return = "<{$tag} class=\"info\">{$description}</{$tag}>" . "\n";
} else {
$return = sprintf('<'.$tag.' class="command">%s</'.$tag.'> - <'.$tag.' class="info">%s</'.$tag.'>',
$commandName, $description) . "\n";
}
return $return;
}
/**
* Arguments tag renderer, arguments is the alias for param tag if
* tag has an alias it should be specified in renderer name instead of
* original tag name. As the result: _renderParamTag => _renderArgumentsTag
*
* @param string $commandName string
* @param array $tags array of available tags
* @param string $tagName given tag name (arguments in this case)
* @return string rendered tag with style placeholders
*/
protected function _renderArgumentsTag($commandName, array $tags, $tagName)
{
$return = '';
if (!array_key_exists($tagName, $tags)) {
return $return;
}
$args = $tags[$tagName];
$padding = $this->_findLongestElementInArray(array_keys($tags));
$tagName = str_pad($tagName, $padding);
$pattern = "/^([^\s]+)\s([^\s]+)\s?(.*)$/e";
$replace = "'--'.str_replace('\$','','\\2').'=<\\1>: \\3'";
foreach ((array)$args as $i => $arg) {
$arg = preg_replace($pattern, $replace, $arg);
if (0 == $i) {
$return .= sprintf(' %s : %s', ucfirst($tagName),
$arg) . "\n";
} else {
$return .= sprintf(' %s %s', str_pad(' ', $padding),
$arg) . "\n";
}
}
return $return;
}
}
| {'content_hash': '97b62aa883659b774e838e929c0c43d6', 'timestamp': '', 'source': 'github', 'line_count': 74, 'max_line_length': 111, 'avg_line_length': 33.445945945945944, 'alnum_prop': 0.5163636363636364, 'repo_name': 'b-b3rn4rd/Terminalor', 'id': '224e84ed592142e650fc0cbd31c48491b6fc1ba8', 'size': '2922', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'library/Terminalor/DocParser/Template/Standard.php', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'PHP', 'bytes': '245829'}]} |
FROM node:slim
COPY . /action
WORKDIR /action
RUN npm install --production
ENTRYPOINT ["node", "/action/main.js"]
| {'content_hash': '99483b1c21ad486ead21a96b02e1e0ad', 'timestamp': '', 'source': 'github', 'line_count': 8, 'max_line_length': 38, 'avg_line_length': 14.625, 'alnum_prop': 0.717948717948718, 'repo_name': 'rust-analyzer/rust-analyzer', 'id': '5849eac7d246a30549ffff371a5e54ae0d099967', 'size': '117', 'binary': False, 'copies': '8', 'ref': 'refs/heads/master', 'path': '.github/actions/github-release/Dockerfile', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'HTML', 'bytes': '173570'}, {'name': 'JavaScript', 'bytes': '1284'}, {'name': 'RenderScript', 'bytes': '45'}, {'name': 'Rust', 'bytes': '8596509'}, {'name': 'Shell', 'bytes': '120'}, {'name': 'TypeScript', 'bytes': '147022'}]} |
Subsets and Splits
No saved queries yet
Save your SQL queries to embed, download, and access them later. Queries will appear here once saved.