text
stringlengths 4
5.48M
| meta
stringlengths 14
6.54k
|
---|---|
* Write a program that prints the entire ASCII table of characters on the console (characters from 0 to 255).
* Note: Some characters have a special purpose and will not be displayed as expected. You may skip them or display them differently. | {'content_hash': '21bdef7d36938f47781bef95e1c3aa8b', 'timestamp': '', 'source': 'github', 'line_count': 3, 'max_line_length': 133, 'avg_line_length': 82.0, 'alnum_prop': 0.7804878048780488, 'repo_name': 'petyakostova/Telerik-Academy', 'id': '0c5719836f45157098c694c2412ffbfa19c11a9c', 'size': '286', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'C#/C# 1/2. PrimitiveDataTypesVariables-Homework/Print-The-ASCII-Table/README.md', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Batchfile', 'bytes': '260'}, {'name': 'C#', 'bytes': '2688607'}, {'name': 'CSS', 'bytes': '165511'}, {'name': 'CoffeeScript', 'bytes': '4962'}, {'name': 'HTML', 'bytes': '6067263'}, {'name': 'JavaScript', 'bytes': '2379763'}]} |
import os
import sys
from common import call_pandoc, regex_process, listfiles
def convert_to_wordpress(filename):
"""Convert from Markdown to Wordpress."""
basename, ext = os.path.splitext(filename)
print("Converting '{0:s}' to Wordpress HTML...".format(filename))
filename1 = basename + ".wordpress_tmp.txt"
call_pandoc(filename, filename1, "markdown", "html", ["--gladtex"])
# postprocessing for wordpress and MathJax-Latex
patterns = [("<EQ ENV=\"math\">(.*?)</EQ>", "[latex]\\1[/latex]"),
("<EQ ENV=\"displaymath\">(.*?)</EQ>", "<div style=\"text-align: center;\">[latex]\\1[/latex]</div>"),
("<pre><code>","<pre lang=\"python\">"),
("</code></pre>","</pre>"),
(""", "\""),
("'", "'"),
("<h1(.*?)>(.*?)</h1>\n", ""),
]
filename2 = basename + ".wordpress.txt"
regex_process(filename1, filename2, patterns)
os.remove(filename1)
print("Done!")
def main():
for file in listfiles():
convert_to_wordpress(file)
if __name__ == '__main__':
main()
| {'content_hash': '4cbc45c6f5c9b836adf35f51d2ba2fc7', 'timestamp': '', 'source': 'github', 'line_count': 31, 'max_line_length': 118, 'avg_line_length': 36.483870967741936, 'alnum_prop': 0.5313881520778072, 'repo_name': 'rossant/mdconvert', 'id': 'd3f2a17941e0a2a0f0a1102adbf04715594237db', 'size': '1131', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'mdconvert/mdwp.py', 'mode': '33188', 'license': 'bsd-3-clause', 'language': [{'name': 'Python', 'bytes': '3732'}]} |
"""
Dijkstra's algorithm implementation
https://en.wikipedia.org/wiki/Dijkstra%27s_algorithm
"""
from __future__ import print_function
def dijkstra(edges, source, target):
""" Dijkstra's algorithm
Parameters
----------
edges : list of tuples
list of dependencies between nodes in the graph
[(source, target, weight), ...]
source : str
name of source node of the graph
target : str
name of target node of the graph
Returns
-------
out : tuple
length and path between source and target node
tuple (length, path)
"""
# determine the graph as dict where:
# key - source node and
# item - list of [(destination, weight), ...]
graph = {}
for src, dest, weight in edges:
if src not in graph:
graph[src] = []
graph[src].append((dest, weight))
# set a queue
# weight, source node, path from source node
queue = [(0, source, [source])]
# initialize visited nodes
# visited nodes - nodes with a known shortest path
# key - visited node
# item - (cost, path)
visited = dict()
while queue:
# get item from queue
cost, src, path = queue.pop()
if src not in visited:
# this is a new visited node
visited[src] = (cost, path)
if src == target:
# target node was reached
return cost, path
# set initial weight, destination node and path
min_weight, min_dest, min_path = float('Inf'), None, None
# loop for all visited nodes
for src_, (cost_, path_) in visited.items():
# find a shortest path
# from src node to the nearest node
for dest, weight in graph.get(src_, ()):
if dest in visited:
# skip nodes with a known shortest path
continue
# calculate current cost
current_cost = cost_ + weight
if current_cost < min_weight:
min_weight, min_dest, min_path = current_cost, dest, path_
if min_dest is not None:
# we find shortest path to new node
# shortest path was updated
path = min_path + [min_dest]
# add to the queue
queue.insert(0, (min_weight, min_dest, path))
# Return infinity if the target node is not reached
return float('Inf'), []
if __name__ == "__main__":
EDGES = [
('s', 't', 6),
('s', 'y', 7),
('t', 'x', 5),
('x', 't', -2),
('t', 'y', 8),
('y', 'z', 9),
('y', 'x', -3),
('t', 'z', -4),
('z', 's', 2),
('z', 'x', 7)
]
for SRC, DEST, MINLEN in [
('s', 'z', 2), ('a', 'z', float('Inf')), ('s', 's', 0)]:
LEN, PATH = dijkstra(EDGES, SRC, DEST)
print("Length of the path from '{}' to '{}' = {}, ({})"
.format(SRC, DEST, LEN, LEN == MINLEN))
print("Path from '{}' to '{}': {}".format(SRC, DEST, PATH))
| {'content_hash': 'c35c242a424f460aa19eecbac1f2d865', 'timestamp': '', 'source': 'github', 'line_count': 101, 'max_line_length': 78, 'avg_line_length': 30.514851485148515, 'alnum_prop': 0.5045425048669695, 'repo_name': 'dsysoev/fun-with-algorithms', 'id': '4996c07338f898bcad5f9b67bb3534898fe28311', 'size': '3084', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'graph/dijkstra.py', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Python', 'bytes': '150209'}]} |
.class final Lo/BZ;
.super Lo/BY;
.source ""
# annotations
.annotation system Ldalvik/annotation/InnerClass;
accessFlags = 0x8
name = null
.end annotation
.annotation system Ldalvik/annotation/Signature;
value = {
"Lo/BY<TK;TV;>;"
}
.end annotation
# instance fields
.field final synthetic ˊ:Lo/BY;
.field final synthetic ˋ:Ljava/util/concurrent/Executor;
# direct methods
.method constructor <init>(Lo/BY;Ljava/util/concurrent/Executor;)V
.locals 0
.line 186
iput-object p1, p0, Lo/BZ;->ˊ:Lo/BY;
iput-object p2, p0, Lo/BZ;->ˋ:Ljava/util/concurrent/Executor;
invoke-direct {p0}, Lo/BY;-><init>()V
return-void
.end method
# virtual methods
.method public ˊ(Ljava/lang/Object;)Ljava/lang/Object;
.locals 1
.annotation system Ldalvik/annotation/Signature;
value = {
"(TK;)TV;"
}
.end annotation
.line 189
iget-object v0, p0, Lo/BZ;->ˊ:Lo/BY;
invoke-virtual {v0, p1}, Lo/BY;->ˊ(Ljava/lang/Object;)Ljava/lang/Object;
move-result-object v0
return-object v0
.end method
.method public ˊ(Ljava/lang/Iterable;)Ljava/util/Map;
.locals 1
.annotation system Ldalvik/annotation/Signature;
value = {
"(Ljava/lang/Iterable<+TK;>;)Ljava/util/Map<TK;TV;>;"
}
.end annotation
.line 206
iget-object v0, p0, Lo/BZ;->ˊ:Lo/BY;
invoke-virtual {v0, p1}, Lo/BY;->ˊ(Ljava/lang/Iterable;)Ljava/util/Map;
move-result-object v0
return-object v0
.end method
.method public ˊ(Ljava/lang/Object;Ljava/lang/Object;)Lo/Rc;
.locals 2
.annotation system Ldalvik/annotation/Signature;
value = {
"(TK;TV;)Lo/Rc<TV;>;"
}
.end annotation
.line 194
new-instance v0, Lo/Ca;
invoke-direct {v0, p0, p1, p2}, Lo/Ca;-><init>(Lo/BZ;Ljava/lang/Object;Ljava/lang/Object;)V
invoke-static {v0}, Lo/Rd;->ˊ(Ljava/util/concurrent/Callable;)Lo/Rd;
move-result-object v1
.line 200
iget-object v0, p0, Lo/BZ;->ˋ:Ljava/util/concurrent/Executor;
invoke-interface {v0, v1}, Ljava/util/concurrent/Executor;->execute(Ljava/lang/Runnable;)V
.line 201
return-object v1
.end method
| {'content_hash': '854daa52071182789e22942339d87aa4', 'timestamp': '', 'source': 'github', 'line_count': 101, 'max_line_length': 95, 'avg_line_length': 21.742574257425744, 'alnum_prop': 0.6429872495446266, 'repo_name': 'gelldur/jak_oni_to_robia_prezentacja', 'id': '7e7f550c502c940b374d4636ab6deea2d151c98c', 'size': '2209', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'Starbucks/output/smali/o/BZ.smali', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Java', 'bytes': '8427'}, {'name': 'Shell', 'bytes': '2303'}, {'name': 'Smali', 'bytes': '57557982'}]} |
var util = require('util');
var plugins = require('../../services/plugins');
var Parent = plugins.require('models/fields/select');
function Checkboxlist() {
Parent.call(this);
this.on('getting.options', function(event) {
if (typeof event.value === 'function') {
event.value = event.value.call(this);
}
});
}
util.inherits(Checkboxlist, Parent);
module.exports = Checkboxlist;
| {'content_hash': '03dcffbba05c2fbf0e0d375c3acef15f', 'timestamp': '', 'source': 'github', 'line_count': 17, 'max_line_length': 54, 'avg_line_length': 24.941176470588236, 'alnum_prop': 0.6297169811320755, 'repo_name': 'spleenboy/pallium-cms', 'id': 'b262873633a0b6c01048a46bba786e1491d01015', 'size': '424', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'app/models/fields/checkboxlist.js', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '231825'}, {'name': 'HTML', 'bytes': '60887'}, {'name': 'JavaScript', 'bytes': '105960'}]} |
package eventstore.j;
import java.io.Closeable;
import java.util.Collection;
import scala.concurrent.Future;
import akka.NotUsed;
import akka.stream.javadsl.Source;
import eventstore.akka.SubscriptionObserver;
import eventstore.core.settings.PersistentSubscriptionSettings;
import eventstore.core.*;
/**
* Maintains a full duplex connection to the EventStore
* <p>
* All operations are handled in a full async manner.
* Many threads can use an <code>EsConnection</code> at the same time or a single thread can make many asynchronous requests.
* To get the most performance out of the connection it is generally recommended to use it in this way.
*/
public interface EsConnection {
/**
* Write events to a stream
* <p>
* When writing events to a stream the {@link eventstore.core.ExpectedVersion} choice can
* make a very large difference in the observed behavior. For example, if no stream exists
* and ExpectedVersion.Any is used, a new stream will be implicitly created when appending.
* <p>
* There are also differences in idempotency between different types of calls.
* If you specify an ExpectedVersion aside from ExpectedVersion.Any the Event Store
* will give you an idempotency guarantee. If using ExpectedVersion.Any the Event Store
* will do its best to provide idempotency but does not guarantee idempotency
*
* @param stream name of the stream to write events to
* @param expectedVersion expected version of the stream to write to, or <code>ExpectedVersion.Any</code> if <code>null</code>
* @param events events to append to the stream
* @param credentials optional user credentials to perform operation with.
* @return A {@link scala.concurrent.Future} that the caller can await on
*/
Future<WriteResult> writeEvents(
String stream,
ExpectedVersion expectedVersion,
Collection<EventData> events,
UserCredentials credentials);
/**
* Write events to a stream
* <p>
* When writing events to a stream the {@link eventstore.core.ExpectedVersion} choice can
* make a very large difference in the observed behavior. For example, if no stream exists
* and ExpectedVersion.Any is used, a new stream will be implicitly created when appending.
* <p>
* There are also differences in idempotency between different types of calls.
* If you specify an ExpectedVersion aside from ExpectedVersion.Any the Event Store
* will give you an idempotency guarantee. If using ExpectedVersion.Any the Event Store
* will do its best to provide idempotency but does not guarantee idempotency
*
* @param stream name of the stream to write events to
* @param expectedVersion expected version of the stream to write to, or <code>ExpectedVersion.Any</code> if <code>null</code>
* @param events events to append to the stream
* @param credentials optional user credentials to perform operation with.
* @param requireMaster Require Event Store to refuse operation if it is not master
* @return A {@link scala.concurrent.Future} that the caller can await on
*/
Future<WriteResult> writeEvents(
String stream,
ExpectedVersion expectedVersion,
Collection<EventData> events,
UserCredentials credentials,
boolean requireMaster);
/**
* Deletes a stream from the Event Store
*
* @param stream name of the stream to delete
* @param expectedVersion optional expected version that the stream should have when being deleted, or <code>ExpectedVersion.Any</code> if <code>null</code>
* @param credentials optional user credentials to perform operation with.
* @return A {@link scala.concurrent.Future} that the caller can await on
*/
Future<DeleteResult> deleteStream(
String stream,
ExpectedVersion.Existing expectedVersion,
UserCredentials credentials);
/**
* Deletes a stream from the Event Store
*
* @param stream name of the stream to delete
* @param expectedVersion optional expected version that the stream should have when being deleted, or <code>ExpectedVersion.Any</code> if <code>null</code>
* @param hardDelete Indicator for tombstoning vs soft-deleting the stream. Tombstoned streams can never be recreated. Soft-deleted streams can be written to again, but the EventNumber sequence will not start from 0.
* @param credentials optional user credentials to perform operation with.
* @return A {@link scala.concurrent.Future} that the caller can await on
*/
Future<DeleteResult> deleteStream(
String stream,
ExpectedVersion.Existing expectedVersion,
boolean hardDelete,
UserCredentials credentials);
/**
* Deletes a stream from the Event Store
*
* @param stream name of the stream to delete
* @param expectedVersion optional expected version that the stream should have when being deleted, or <code>ExpectedVersion.Any</code> if <code>null</code>
* @param hardDelete Indicator for tombstoning vs soft-deleting the stream. Tombstoned streams can never be recreated. Soft-deleted streams can be written to again, but the EventNumber sequence will not start from 0.
* @param credentials optional user credentials to perform operation with.
* @param requireMaster Require Event Store to refuse operation if it is not master
* @return A {@link scala.concurrent.Future} that the caller can await on
*/
Future<DeleteResult> deleteStream(
String stream,
ExpectedVersion.Existing expectedVersion,
boolean hardDelete,
UserCredentials credentials,
boolean requireMaster);
/**
* Starts a transaction in the event store on a given stream asynchronously
* <p>
* A {@link eventstore.j.EsTransaction} allows the calling of multiple writes with multiple
* round trips over long periods of time between the caller and the event store. This method
* is only available through the TCP interface and no equivalent exists for the RESTful interface.
*
* @param stream The stream to start a transaction on
* @param expectedVersion The expected version of the stream at the time of starting the transaction
* @param credentials The optional user credentials to perform operation with
* @return A {@link scala.concurrent.Future} containing an actual transaction
*/
Future<EsTransaction> startTransaction(
String stream,
ExpectedVersion expectedVersion,
UserCredentials credentials);
/**
* Starts a transaction in the event store on a given stream asynchronously
* <p>
* A {@link eventstore.j.EsTransaction} allows the calling of multiple writes with multiple
* round trips over long periods of time between the caller and the event store. This method
* is only available through the TCP interface and no equivalent exists for the RESTful interface.
*
* @param stream The stream to start a transaction on
* @param expectedVersion The expected version of the stream at the time of starting the transaction
* @param credentials The optional user credentials to perform operation with
* @param requireMaster Require Event Store to refuse operation if it is not master
* @return A {@link scala.concurrent.Future} containing an actual transaction
*/
Future<EsTransaction> startTransaction(
String stream,
ExpectedVersion expectedVersion,
UserCredentials credentials,
boolean requireMaster);
/**
* Continues transaction by provided transaction ID.
* <p>
* A {@link eventstore.j.EsTransaction} allows the calling of multiple writes with multiple
* round trips over long periods of time between the caller and the event store. This method
* is only available through the TCP interface and no equivalent exists for the RESTful interface.
*
* @param transactionId The transaction ID that needs to be continued.
* @param credentials The optional user credentials to perform operation with
* @return A transaction for given id
*/
EsTransaction continueTransaction(long transactionId, UserCredentials credentials);
/**
* Reads a single event from a stream at event number
*
* @param stream name of the stream to read from
* @param eventNumber optional event number to read, or EventNumber.Last for reading latest event, EventNumber.Last if null
* @param resolveLinkTos whether to resolve LinkTo events automatically
* @param credentials The optional user credentials to perform operation with
* @return A {@link scala.concurrent.Future} containing an event
*/
Future<Event> readEvent(
String stream,
EventNumber eventNumber,
boolean resolveLinkTos,
UserCredentials credentials);
/**
* Reads a single event from a stream at event number
*
* @param stream name of the stream to read from
* @param eventNumber optional event number to read, or EventNumber.Last for reading latest event, EventNumber.Last if null
* @param resolveLinkTos whether to resolve LinkTo events automatically
* @param credentials The optional user credentials to perform operation with
* @param requireMaster Require Event Store to refuse operation if it is not master
* @return A {@link scala.concurrent.Future} containing an event
*/
Future<Event> readEvent(
String stream,
EventNumber eventNumber,
boolean resolveLinkTos,
UserCredentials credentials,
boolean requireMaster);
/**
* Reads count events from a stream forwards (e.g. oldest to newest) starting from event number
*
* @param stream name of stream to read from
* @param fromNumber optional event number to read, EventNumber.First if null
* @param maxCount maximum count of items to read
* @param resolveLinkTos whether to resolve LinkTo events automatically
* @param credentials The optional user credentials to perform operation with
* @return A {@link scala.concurrent.Future} containing the results of the read operation
*/
Future<ReadStreamEventsCompleted> readStreamEventsForward(
String stream,
EventNumber.Exact fromNumber,
int maxCount,
boolean resolveLinkTos,
UserCredentials credentials);
/**
* Reads count events from a stream forwards (e.g. oldest to newest) starting from event number
*
* @param stream name of stream to read from
* @param fromNumber optional event number to read, EventNumber.First if null
* @param maxCount maximum count of items to read
* @param resolveLinkTos whether to resolve LinkTo events automatically
* @param credentials The optional user credentials to perform operation with
* @param requireMaster Require Event Store to refuse operation if it is not master
* @return A {@link scala.concurrent.Future} containing the results of the read operation
*/
Future<ReadStreamEventsCompleted> readStreamEventsForward(
String stream,
EventNumber.Exact fromNumber,
int maxCount,
boolean resolveLinkTos,
UserCredentials credentials,
boolean requireMaster);
/**
* Reads count events from from a stream backwards (e.g. newest to oldest) starting from event number
*
* @param stream name of stream to read from
* @param fromNumber optional event number to read, EventNumber.Last if null
* @param maxCount maximum count of items to read
* @param resolveLinkTos whether to resolve LinkTo events automatically
* @param credentials The optional user credentials to perform operation with
* @return A {@link scala.concurrent.Future} containing the results of the read operation
*/
Future<ReadStreamEventsCompleted> readStreamEventsBackward(
String stream,
EventNumber fromNumber,
int maxCount,
boolean resolveLinkTos,
UserCredentials credentials);
/**
* Reads count events from from a stream backwards (e.g. newest to oldest) starting from event number
*
* @param stream name of stream to read from
* @param fromNumber optional event number to read, EventNumber.Last if null
* @param maxCount maximum count of items to read
* @param resolveLinkTos whether to resolve LinkTo events automatically
* @param credentials The optional user credentials to perform operation with
* @param requireMaster Require Event Store to refuse operation if it is not master
* @return A {@link scala.concurrent.Future} containing the results of the read operation
*/
Future<ReadStreamEventsCompleted> readStreamEventsBackward(
String stream,
EventNumber fromNumber,
int maxCount,
boolean resolveLinkTos,
UserCredentials credentials,
boolean requireMaster);
/**
* Reads all events in the node forward (e.g. beginning to end) starting from position
*
* @param fromPosition optional position to start reading from, Position.First of null
* @param maxCount maximum count of items to read
* @param resolveLinkTos whether to resolve LinkTo events automatically
* @param credentials The optional user credentials to perform operation with
* @return A {@link scala.concurrent.Future} containing the results of the read operation
*/
Future<ReadAllEventsCompleted> readAllEventsForward(
Position fromPosition,
int maxCount,
boolean resolveLinkTos,
UserCredentials credentials);
/**
* Reads all events in the node forward (e.g. beginning to end) starting from position
*
* @param fromPosition optional position to start reading from, Position.First of null
* @param maxCount maximum count of items to read
* @param resolveLinkTos whether to resolve LinkTo events automatically
* @param credentials The optional user credentials to perform operation with
* @param requireMaster Require Event Store to refuse operation if it is not master
* @return A {@link scala.concurrent.Future} containing the results of the read operation
*/
Future<ReadAllEventsCompleted> readAllEventsForward(
Position fromPosition,
int maxCount,
boolean resolveLinkTos,
UserCredentials credentials,
boolean requireMaster);
/**
* Reads all events in the node backwards (e.g. end to beginning) starting from position
*
* @param fromPosition optional position to start reading from, Position.Last of null
* @param maxCount maximum count of items to read
* @param resolveLinkTos whether to resolve LinkTo events automatically
* @param credentials The optional user credentials to perform operation with
* @return A {@link scala.concurrent.Future} containing the results of the read operation
*/
Future<ReadAllEventsCompleted> readAllEventsBackward(
Position fromPosition,
int maxCount,
boolean resolveLinkTos,
UserCredentials credentials);
/**
* Reads all events in the node backwards (e.g. end to beginning) starting from position
*
* @param fromPosition optional position to start reading from, Position.Last of null
* @param maxCount maximum count of items to read
* @param resolveLinkTos whether to resolve LinkTo events automatically
* @param credentials The optional user credentials to perform operation with
* @param requireMaster Require Event Store to refuse operation if it is not master
* @return A {@link scala.concurrent.Future} containing the results of the read operation
*/
Future<ReadAllEventsCompleted> readAllEventsBackward(
Position fromPosition,
int maxCount,
boolean resolveLinkTos,
UserCredentials credentials,
boolean requireMaster);
/**
* Subscribes to a single event stream. New events
* written to the stream while the subscription is active will be
* pushed to the client.
*
* @param stream The stream to subscribe to
* @param observer A {@link eventstore.akka.SubscriptionObserver} to handle a new event received over the subscription
* @param resolveLinkTos Whether to resolve LinkTo events automatically
* @param credentials The optional user credentials to perform operation with
* @return A {@link java.io.Closeable} representing the subscription which can be closed.
*/
Closeable subscribeToStream(
String stream,
SubscriptionObserver<Event> observer,
boolean resolveLinkTos,
UserCredentials credentials);
/**
* Subscribes to a single event stream. Existing events from
* lastCheckpoint onwards are read from the stream
* and presented to the user of <code>SubscriptionObserver</code>
* as if they had been pushed.
* <p>
* Once the end of the stream is read the subscription is
* transparently (to the user) switched to push new events as
* they are written.
* <p>
* If events have already been received and resubscription from the same point
* is desired, use the event number of the last event processed which
* appeared on the subscription.
*
* @param stream The stream to subscribe to
* @param observer A {@link eventstore.akka.SubscriptionObserver} to handle a new event received over the subscription
* @param fromEventNumberExclusive The event number from which to start, or <code>null</code> to read all events.
* @param resolveLinkTos Whether to resolve LinkTo events automatically
* @param credentials The optional user credentials to perform operation with
* @return A {@link java.io.Closeable} representing the subscription which can be closed.
*/
Closeable subscribeToStreamFrom(
String stream,
SubscriptionObserver<Event> observer,
Long fromEventNumberExclusive,
boolean resolveLinkTos,
UserCredentials credentials);
/**
* Subscribes to all events in the Event Store. New events written to the stream
* while the subscription is active will be pushed to the client.
*
* @param observer A {@link eventstore.akka.SubscriptionObserver} to handle a new event received over the subscription
* @param resolveLinkTos Whether to resolve LinkTo events automatically
* @param credentials The optional user credentials to perform operation with
* @return A {@link java.io.Closeable} representing the subscription which can be closed.
*/
Closeable subscribeToAll(
SubscriptionObserver<IndexedEvent> observer,
boolean resolveLinkTos,
UserCredentials credentials);
/**
* Subscribes to a all events. Existing events from position
* onwards are read from the Event Store and presented to the user of
* <code>SubscriptionObserver</code> as if they had been pushed.
* <p>
* Once the end of the stream is read the subscription is
* transparently (to the user) switched to push new events as
* they are written.
* <p>
* If events have already been received and resubscription from the same point
* is desired, use the position representing the last event processed which
* appeared on the subscription.
*
* @param observer A {@link eventstore.akka.SubscriptionObserver} to handle a new event received over the subscription
* @param fromPositionExclusive The position from which to start, or <code>null</code> to read all events
* @param resolveLinkTos Whether to resolve LinkTo events automatically
* @param credentials The optional user credentials to perform operation with
* @return A {@link java.io.Closeable} representing the subscription which can be closed.
*/
Closeable subscribeToAllFrom(
SubscriptionObserver<IndexedEvent> observer,
Position.Exact fromPositionExclusive,
boolean resolveLinkTos,
UserCredentials credentials);
// TODO support stream not found
// Future<Unit> setStreamMetadata(String stream, int expectedMetastreamVersion, StreamMetadata metadata, UserCredentials credentials);
// Future<StreamMetadataResult> getStreamMetadataAsync(String stream, UserCredentials credentials);
// Future<RawStreamMetadataResult> getStreamMetadataAsRawBytesAsync(String stream, UserCredentials credentials); TODO
/**
* Sets the metadata for a stream.
*
* @param stream The name of the stream for which to set metadata.
* @param expectedMetastreamVersion The expected version for the write to the metadata stream.
* @param metadata A byte array representing the new metadata.
* @param credentials The optional user credentials to perform operation with
* @return A {@link scala.concurrent.Future} representing the operation
*/
Future<WriteResult> setStreamMetadata(
String stream,
ExpectedVersion expectedMetastreamVersion,
byte[] metadata,
UserCredentials credentials);
/**
* Reads the metadata for a stream as a byte array.
*
* @param stream The name of the stream for which to read metadata.
* @param credentials The optional user credentials to perform operation with
* @return A {@link scala.concurrent.Future} containing the metadata as byte array.
*/
Future<byte[]> getStreamMetadataBytes(String stream, UserCredentials credentials);
/**
* Creates a Source you can use to subscribe to a single event stream. Existing events from
* event number onwards are read from the stream and presented to the user of
* <code>Source</code> as if they had been pushed.
* <p>
* Once the end of the stream is read the <code>Source</code> transparently (to the user)
* switches to push new events as they are written.
* <p>
* If events have already been received and resubscription from the same point
* is desired, use the event number of the last event processed.
*
* @param stream The stream to subscribe to
* @param fromEventNumberExclusive The event number from which to start, or <code>null</code> to read all events.
* @param resolveLinkTos Whether to resolve LinkTo events automatically
* @param credentials The optional user credentials to perform operation with
* @param infinite Whether to subscribe to the future events upon reading all current
* @return A {@link akka.stream.javadsl.Source} representing the stream
*/
Source<Event, NotUsed> streamSource(
String stream,
EventNumber fromEventNumberExclusive,
boolean resolveLinkTos,
UserCredentials credentials,
boolean infinite);
/**
* Creates a Source you can use to subscribes to all events. Existing events from position
* onwards are read from the Event Store and presented to the user of
* <code>Source</code> as if they had been pushed.
* <p>
* Once the end of the stream is read the <code>Source</code> transparently (to the user)
* switches to push new events as they are written.
* <p>
* If events have already been received and resubscription from the same point
* is desired, use the position representing the last event processed.
*
* @param fromPositionExclusive The position from which to start, or <code>null</code> to read all events
* @param resolveLinkTos Whether to resolve LinkTo events automatically
* @param credentials The optional user credentials to perform operation with
* @param infinite Whether to subscribe to the future events upon reading all current
* @return A {@link akka.stream.javadsl.Source} representing all streams
*/
Source<IndexedEvent, NotUsed> allStreamsSource(
Position fromPositionExclusive,
boolean resolveLinkTos,
UserCredentials credentials,
boolean infinite);
/**
* Asynchronously create a persistent subscription group on a stream
*
* @param stream The name of the stream to create the persistent subscription on
* @param groupName The name of the group to create
* @param settings The {@link PersistentSubscriptionSettings} for the subscription, or <code>null</code> for defaults
* @param credentials The credentials to be used for this operation
* @return A {@link scala.concurrent.Future} representing the operation
*/
Future<scala.Unit> createPersistentSubscription(
String stream,
String groupName,
PersistentSubscriptionSettings settings,
UserCredentials credentials);
/**
* Asynchronously update a persistent subscription group on a stream
*
* @param stream The name of the stream to create the persistent subscription on
* @param groupName The name of the group to create
* @param settings The {@link PersistentSubscriptionSettings} for the subscription, or <code>null</code> for defaults
* @param credentials The credentials to be used for this operation, or <code>null</code> for default
* @return A {@link scala.concurrent.Future} representing the operation
*/
Future<scala.Unit> updatePersistentSubscription(
String stream,
String groupName,
PersistentSubscriptionSettings settings,
UserCredentials credentials);
/**
* Asynchronously delete a persistent subscription group on a stream
*
* @param stream The name of the stream to create the persistent subscription on
* @param groupName The name of the group to create
* @param credentials The credentials to be used for this operation, or <code>null</code> for default
* @return A {@link scala.concurrent.Future} representing the operation
*/
Future<scala.Unit> deletePersistentSubscription(
String stream,
String groupName,
UserCredentials credentials);
}
| {'content_hash': '013f65b93d0485b19f4c49371bbf21a9', 'timestamp': '', 'source': 'github', 'line_count': 547, 'max_line_length': 223, 'avg_line_length': 47.09140767824497, 'alnum_prop': 0.7269303932606079, 'repo_name': 'EventStore/EventStore.JVM', 'id': 'e3379f754431c44dcddde909313919f9abafe6b0', 'size': '25759', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'client/src/main/java/eventstore/j/EsConnection.java', 'mode': '33188', 'license': 'bsd-3-clause', 'language': [{'name': 'Java', 'bytes': '29794'}, {'name': 'Scala', 'bytes': '683287'}]} |
ACCEPTED
#### According to
International Plant Names Index
#### Published in
null
#### Original name
null
### Remarks
null | {'content_hash': '8f008fea1cd386dbe0af5a045b6395f5', 'timestamp': '', 'source': 'github', 'line_count': 13, 'max_line_length': 31, 'avg_line_length': 9.692307692307692, 'alnum_prop': 0.7063492063492064, 'repo_name': 'mdoering/backbone', 'id': '88b59771f656bf3074ccb58259c5afc1d4b908ef', 'size': '174', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'life/Plantae/Magnoliophyta/Magnoliopsida/Lamiales/Lamiaceae/Sideritis/Sideritis rigida/README.md', 'mode': '33188', 'license': 'apache-2.0', 'language': []} |
<h2>Editing <span class='muted'>OilColor</span></h2>
<br>
<?php echo render('oilcolors/_form'); ?>
<p>
<?php echo Html::anchor('oilcolors/view/'.$oilColor->id, 'View'); ?> |
<?php echo Html::anchor('oilcolors', 'Back'); ?></p>
| {'content_hash': '5295acdbafa7f5409c952f7536d60d8f', 'timestamp': '', 'source': 'github', 'line_count': 7, 'max_line_length': 71, 'avg_line_length': 32.857142857142854, 'alnum_prop': 0.6130434782608696, 'repo_name': 'grantelgin/sw1', 'id': 'a75626d20bdda7fd085f88f39c1f6329efdb039b', 'size': '230', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'fuel/app/views/oilcolors/edit.php', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '155505'}, {'name': 'JavaScript', 'bytes': '58458'}, {'name': 'PHP', 'bytes': '1939677'}]} |
<?php declare(strict_types=1);
namespace Monolog\Handler;
use Monolog\Logger;
use Swift;
use Swift_Message;
/**
* MandrillHandler uses cURL to send the emails to the Mandrill API
*
* @author Adam Nicholson <[email protected]>
*/
class MandrillHandler extends MailHandler
{
/** @var Swift_Message */
protected $message;
/** @var string */
protected $apiKey;
/**
* @psalm-param Swift_Message|callable(): Swift_Message $message
*
* @param string $apiKey A valid Mandrill API key
* @param callable|Swift_Message $message An example message for real messages, only the body will be replaced
*/
public function __construct(string $apiKey, $message, $level = Logger::ERROR, bool $bubble = true)
{
parent::__construct($level, $bubble);
if (!$message instanceof Swift_Message && is_callable($message)) {
$message = $message();
}
if (!$message instanceof Swift_Message) {
throw new \InvalidArgumentException('You must provide either a Swift_Message instance or a callable returning it');
}
$this->message = $message;
$this->apiKey = $apiKey;
}
/**
* {@inheritdoc}
*/
protected function send(string $content, array $records): void
{
$mime = 'text/plain';
if ($this->isHtmlBody($content)) {
$mime = 'text/html';
}
$message = clone $this->message;
$message->setBody($content, $mime);
/** @phpstan-ignore-next-line */
if (version_compare(Swift::VERSION, '6.0.0', '>=')) {
$message->setDate(new \DateTimeImmutable());
} else {
/** @phpstan-ignore-next-line */
$message->setDate(time());
}
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, 'https://mandrillapp.com/api/1.0/messages/send-raw.json');
curl_setopt($ch, CURLOPT_POST, 1);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($ch, CURLOPT_POSTFIELDS, http_build_query([
'key' => $this->apiKey,
'raw_message' => (string) $message,
'async' => false,
]));
Curl\Util::execute($ch);
}
}
| {'content_hash': 'd3cfe461dd487bf3baa6a91775233d4b', 'timestamp': '', 'source': 'github', 'line_count': 76, 'max_line_length': 127, 'avg_line_length': 29.55263157894737, 'alnum_prop': 0.57479964381122, 'repo_name': 'tsugiproject/tsugi', 'id': 'f49dc1c4c3f2c120689306d5ce62e402593636af', 'size': '2473', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'vendor/monolog/monolog/src/Monolog/Handler/MandrillHandler.php', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Dockerfile', 'bytes': '263'}, {'name': 'HTML', 'bytes': '207'}, {'name': 'Hack', 'bytes': '3516'}, {'name': 'Handlebars', 'bytes': '4940'}, {'name': 'JavaScript', 'bytes': '14832'}, {'name': 'PHP', 'bytes': '680440'}, {'name': 'Shell', 'bytes': '524'}]} |
Tucnak pedestrian crossing
| {'content_hash': 'e46f90af307ce107bca7e6a245feca09', 'timestamp': '', 'source': 'github', 'line_count': 2, 'max_line_length': 26, 'avg_line_length': 15.0, 'alnum_prop': 0.8, 'repo_name': 'MichalDM/hello-world', 'id': 'fce0ef58383ff0b62d95b307b42e34639023cb0a', 'size': '40', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'animals/tucnak.md', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '672'}]} |
// # Ghost Editor
//
// Ghost Editor contains a set of modules which make up the editor component
// It manages the left and right panes, and all of the communication between them
// Including scrolling,
/*global document, $, _, Ghost */
(function () {
'use strict';
var Editor = function () {
var self = this,
$document = $(document),
// Create all the needed editor components, passing them what they need to function
markdown = new Ghost.Editor.MarkdownEditor(),
uploadMgr = new Ghost.Editor.UploadManager(markdown),
preview = new Ghost.Editor.HTMLPreview(markdown, uploadMgr),
scrollHandler = new Ghost.Editor.ScrollHandler(markdown, preview),
unloadDirtyMessage,
handleChange,
handleDrag;
unloadDirtyMessage = function () {
return '==============================\n\n' +
'Hey there! It looks like you\'re in the middle of writing' +
' something and you haven\'t saved all of your content.' +
'\n\nSave before you go!\n\n' +
'==============================';
};
handleChange = function () {
self.setDirty(true);
preview.update();
};
handleDrag = function (e) {
e.preventDefault();
};
// Public API
_.extend(this, {
enable: function () {
// Listen for changes
$document.on('markdownEditorChange', handleChange);
// enable editing and scrolling
markdown.enable();
scrollHandler.enable();
},
disable: function () {
// Don't listen for changes
$document.off('markdownEditorChange', handleChange);
// disable editing and scrolling
markdown.disable();
scrollHandler.disable();
},
// Get the markdown value from the editor for saving
// Upload manager makes sure the upload markers are removed beforehand
value: function () {
return uploadMgr.value();
},
setDirty: function (dirty) {
window.onbeforeunload = dirty ? unloadDirtyMessage : null;
}
});
// Initialise
$document.on('drop dragover', handleDrag);
preview.update();
this.enable();
};
Ghost.Editor = Ghost.Editor || {};
Ghost.Editor.Main = Editor;
}()); | {'content_hash': '566dacf6db9747d7f24f7b77ff1b0577', 'timestamp': '', 'source': 'github', 'line_count': 79, 'max_line_length': 91, 'avg_line_length': 33.64556962025316, 'alnum_prop': 0.5052671181339353, 'repo_name': 'xhhjin/heroku-ghost', 'id': '0420d6a694e7c1a45700dc47e26d17f4610c55b9', 'size': '2658', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'core/client/assets/lib/editor/index.js', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '165743'}, {'name': 'Handlebars', 'bytes': '51482'}, {'name': 'JavaScript', 'bytes': '726644'}]} |
<?php
declare(strict_types=1);
namespace whatwedo\TableBundle\Tests\App\Factory;
use whatwedo\TableBundle\Tests\App\Entity\Person;
use whatwedo\TableBundle\Tests\App\Repository\PersonRepository;
use Zenstruck\Foundry\ModelFactory;
use Zenstruck\Foundry\Proxy;
use Zenstruck\Foundry\RepositoryProxy;
/**
* @method static Person|Proxy createOne(array $attributes = [])
* @method static Person[]|Proxy[] createMany(int $number, $attributes = [])
* @method static Person|Proxy find($criteria)
* @method static Person|Proxy findOrCreate(array $attributes)
* @method static Person|Proxy first(string $sortedField = 'id')
* @method static Person|Proxy last(string $sortedField = 'id')
* @method static Person|Proxy random(array $attributes = [])
* @method static Person|Proxy randomOrCreate(array $attributes = [])
* @method static Person[]|Proxy[] all()
* @method static Person[]|Proxy[] findBy(array $attributes)
* @method static Person[]|Proxy[] randomSet(int $number, array $attributes = [])
* @method static Person[]|Proxy[] randomRange(int $min, int $max, array $attributes = [])
* @method static PersonRepository|RepositoryProxy repository()
* @method Person|Proxy create($attributes = [])
*/
final class PersonFactory extends ModelFactory
{
protected function getDefaults(): array
{
return [
'name' => self::faker()->name(),
];
}
protected static function getClass(): string
{
return Person::class;
}
}
| {'content_hash': '9a5dcb2c8cb474119322fbaa77eca63a', 'timestamp': '', 'source': 'github', 'line_count': 42, 'max_line_length': 96, 'avg_line_length': 37.19047619047619, 'alnum_prop': 0.6722151088348272, 'repo_name': 'whatwedo/TableBundle', 'id': 'fa0cf188cbcc3e04810ae429fddf116f1bfb6207', 'size': '1562', 'binary': False, 'copies': '1', 'ref': 'refs/heads/1.0-dev', 'path': 'tests/App/Factory/PersonFactory.php', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '3877'}, {'name': 'JavaScript', 'bytes': '28280'}, {'name': 'Makefile', 'bytes': '749'}, {'name': 'PHP', 'bytes': '183340'}, {'name': 'SCSS', 'bytes': '1381'}, {'name': 'Twig', 'bytes': '76123'}]} |
package com.francetelecom.clara.cloud.presentation.applications;
import com.francetelecom.clara.cloud.core.service.exception.ObjectNotFoundException;
import com.francetelecom.clara.cloud.coremodel.Application;
import com.francetelecom.clara.cloud.presentation.common.AjaxFallbackCustomDataTable;
import com.francetelecom.clara.cloud.presentation.common.PageTemplate;
import com.francetelecom.clara.cloud.presentation.resource.CacheActivatedImage;
import com.francetelecom.clara.cloud.presentation.tools.ApplicationProvider;
import com.francetelecom.clara.cloud.presentation.tools.BusinessExceptionHandler;
import com.francetelecom.clara.cloud.presentation.tools.WicketSession;
import org.apache.wicket.ajax.AjaxRequestTarget;
import org.apache.wicket.ajax.AjaxSelfUpdatingTimerBehavior;
import org.apache.wicket.ajax.markup.html.form.AjaxCheckBox;
import org.apache.wicket.behavior.AttributeAppender;
import org.apache.wicket.extensions.markup.html.repeater.data.grid.ICellPopulator;
import org.apache.wicket.extensions.markup.html.repeater.data.table.AbstractColumn;
import org.apache.wicket.extensions.markup.html.repeater.data.table.IColumn;
import org.apache.wicket.extensions.markup.html.repeater.data.table.PropertyColumn;
import org.apache.wicket.markup.html.WebMarkupContainer;
import org.apache.wicket.markup.html.form.CheckBox;
import org.apache.wicket.markup.html.form.Form;
import org.apache.wicket.markup.html.form.SubmitLink;
import org.apache.wicket.markup.html.form.TextField;
import org.apache.wicket.markup.html.panel.Panel;
import org.apache.wicket.markup.repeater.Item;
import org.apache.wicket.model.*;
import org.apache.wicket.util.time.Duration;
import java.util.ArrayList;
import java.util.List;
/**
* ApplicationsTablePanel
* Panel which show the applications
*
* User: Thomas Escalle - tawe8231
* Entity : FT/OLNC/RD/MAPS/MEP/MSE
* Updated : $LastChangedDate$
* @author : $Author$
* @version : $Revision$
*/
public class ApplicationsTablePanel extends Panel {
private static final long serialVersionUID = 527385503166750788L;
//private static final transient org.slf4j.Logger logger = LoggerFactory.getLogger(ApplicationsTablePanel.class);
AjaxFallbackCustomDataTable<Application, String> dataTable;
CheckBox viewAllCheckBox;
private ApplicationsPage parentPage;
private List<Application> applicationsList;
private Form<?> searchCriteriaForm;
private String searchCriteria;
private WebMarkupContainer refreshContainer;
public ApplicationsTablePanel(String id) {
super(id);
initComponents();
}
private void initComponents() {
refreshContainer = new WebMarkupContainer("refreshApplication");
add(refreshContainer.setOutputMarkupId(true));
}
private StringResourceModel getStringResourceModel(java.lang.String key) {
// BVA fix Localizer warning : cf. https://issues.apache.org/jira/browse/WICKET-990
return new StringResourceModel(key, this, null);
}
private void initTable(){
final List<IColumn<Application, String>> columns = new ArrayList<IColumn<Application, String>>();
columns.add(new PropertyColumn<Application, String>(getStringResourceModel("portal.application.table.header.name"), "label", "label") {
private static final long serialVersionUID = -5548238873027346512L;
@Override
public void populateItem(Item<ICellPopulator<Application>> cellItem, String id, IModel<Application> appIModel) {
cellItem.add(new ApplicationDetailsLinkPanel(id, appIModel));
cellItem.add(new AttributeAppender("title", appIModel.getObject().getLabel()));
}
@Override
public String getCssClass() {
return "label ellipsis";
}
});
columns.add(new PropertyColumn<Application, String>(getStringResourceModel("portal.application.table.header.appCode"), "code", "code") {
private static final long serialVersionUID = 2743741360422459728L;
@Override
public void populateItem(Item<ICellPopulator<Application>> item, String componentId, IModel<Application> rowModel) {
super.populateItem(item, componentId, rowModel);
item.add(new AttributeAppender("title", rowModel.getObject().getCode()));
}
@Override
public String getCssClass() {
return "label ellipsis";
}
});
columns.add(new PropertyColumn<Application, String>(getStringResourceModel("portal.application.table.header.description"), "description", "description"));
columns.add(new PropertyColumn<Application, String>(getStringResourceModel("portal.application.table.header.visibility"), "visibility", "visibility") {
private static final long serialVersionUID = -2362613895284301548L;
@Override
public void populateItem(Item<ICellPopulator<Application>> iCellPopulatorItem, String componentId, IModel<Application> appIModel) {
iCellPopulatorItem.add(new ApplicationVisibilityPanel(componentId, appIModel));
}
@Override
public String getCssClass() {
return "actionShort actionCenter";
}
});
columns.add(new AbstractColumn<Application, String>(getStringResourceModel("portal.application.table.header.actions")) {
private static final long serialVersionUID = -2548831025324697929L;
@Override
public void populateItem(Item<ICellPopulator<Application>> iCellPopulatorItem, String componentId, IModel<Application> appIModel) {
ApplicationActionPanel appActPanel = null;
try {
appActPanel = new ApplicationActionPanel(componentId, appIModel, parentPage.getManageApplication().canBeDeleted(appIModel.getObject().getUID()));
} catch (ObjectNotFoundException e) {
BusinessExceptionHandler handler = new BusinessExceptionHandler(parentPage);
handler.error(e);
}
iCellPopulatorItem.add(appActPanel);
}
@Override
public String getCssClass() {
return "actionShort actionCenter";
}
});
//ApplicationProvider appProv = new ApplicationProvider(this, viewAllCheckBox.getModel(), parentPage.getManageApplication());
ApplicationProvider appProv = new ApplicationProvider(searchCriteria, applicationsList);
dataTable = new AjaxFallbackCustomDataTable<>("applicationsDataTable",columns,appProv, PageTemplate.ROWS_PER_PAGE);
dataTable.add(new AjaxSelfUpdatingTimerBehavior(Duration.seconds(60)));
addOrReplace(dataTable);
}
@Override
protected void onBeforeRender() {
this.parentPage = (ApplicationsPage) getPage();
createSearchCriteriaForm();
viewAllCheckBox = new AjaxCheckBox("allAppsCheckbox", new Model<Boolean>(WicketSession.get().getViewAll())) {
private static final long serialVersionUID = -336651607302799133L;
@Override
protected void onUpdate(AjaxRequestTarget target) {
WicketSession.get().setViewAll(getModelObject());
getApplicationsFromDB();
//
initTable();
refreshContainer.replace(dataTable);
target.add(refreshContainer);
}
};
searchCriteriaForm.addOrReplace(viewAllCheckBox);
getApplicationsFromDB();
initTable();
refreshContainer.addOrReplace(dataTable) ;
super.onBeforeRender();
}
private void getApplicationsFromDB() {
applicationsList = new ArrayList<Application>();
if (viewAllCheckBox.getModelObject()) {
applicationsList = parentPage.getManageApplication().findApplications();
} else {
applicationsList = parentPage.getManageApplication().findMyApplications();
}
}
private void createSearchCriteriaForm() {
searchCriteriaForm = new Form<Void>("searchCriteriaForm");
searchCriteriaForm.add(new CacheActivatedImage("imageHelp.searchField", new ResourceModel("image.help").getObject()));
addOrReplace(searchCriteriaForm);
searchCriteriaForm.add(new TextField<String>("searchCriteria", new PropertyModel<String>(this, "searchCriteria")));
SubmitLink searchLink = new SubmitLink("searchLink");
CacheActivatedImage imageSearch = new CacheActivatedImage("imageSearch", new ResourceModel("image.help").getObject());
searchLink.add(imageSearch);
searchCriteriaForm.add(searchLink);
}
}
| {'content_hash': '5e4201c3ca1a1cb61a4fb2b5219cb6cd', 'timestamp': '', 'source': 'github', 'line_count': 212, 'max_line_length': 165, 'avg_line_length': 41.405660377358494, 'alnum_prop': 0.7058555479608111, 'repo_name': 'Orange-OpenSource/elpaaso-core', 'id': '08c077050c64103259b2829f67e856fe050ae781', 'size': '9359', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'cloud-paas/cloud-paas-webapp/cloud-paas-webapp-war/src/main/java/com/francetelecom/clara/cloud/presentation/applications/ApplicationsTablePanel.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Batchfile', 'bytes': '3983'}, {'name': 'CSS', 'bytes': '78369'}, {'name': 'Cucumber', 'bytes': '55546'}, {'name': 'HTML', 'bytes': '170257'}, {'name': 'Java', 'bytes': '4183310'}, {'name': 'JavaScript', 'bytes': '5958'}]} |
import { Routes } from '@angular/router';
import { HomeComponent } from './home/home.component';
import { ProfileComponent } from './profile/profile.component';
import { CallbackComponent } from './callback/callback.component';
// import { DashboardComponent } from './dashboard/dashboard.component';
// import { BootstrapComponent } from './bootstrap/bootstrap-component.component';
import { Error404Component } from './errors/error404.component';
export const ROUTES: Routes = [
{ path: '', component: HomeComponent },
{ path: 'dashboard', component: Error404Component },
{ path: 'bootstrap/:id', component: Error404Component },
{ path: 'profile', component: ProfileComponent },
{ path: 'callback', component: CallbackComponent },
{ path: '**', redirectTo: '' }
];
| {'content_hash': 'db877e6da6abe0a8ec0e170894d9c976', 'timestamp': '', 'source': 'github', 'line_count': 16, 'max_line_length': 83, 'avg_line_length': 48.9375, 'alnum_prop': 0.7100893997445722, 'repo_name': 'jackhummah/bootles', 'id': 'ec0dda5376d066c31372035fd09e10b5ca7cade8', 'size': '783', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/app/app.routes.ts', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '642692'}, {'name': 'HTML', 'bytes': '476397'}, {'name': 'JavaScript', 'bytes': '360085'}, {'name': 'TypeScript', 'bytes': '16808'}]} |
/* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.activiti.engine.impl.persistence.entity;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.activiti.engine.impl.EventSubscriptionQueryImpl;
import org.activiti.engine.impl.Page;
import org.activiti.engine.impl.persistence.AbstractManager;
/**
* @author Daniel Meyer
*/
public class EventSubscriptionManager extends AbstractManager {
/** keep track of subscriptions created in the current command */
protected List<EventSubscriptionEntity> createdSignalSubscriptions = new ArrayList<EventSubscriptionEntity>();
public void insert(EventSubscriptionEntity persistentObject) {
super.insert(persistentObject);
if(persistentObject instanceof SignalEventSubscriptionEntity) {
createdSignalSubscriptions.add(persistentObject);
}
}
public void deleteEventSubscription(EventSubscriptionEntity persistentObject) {
getDbSqlSession().delete(persistentObject.getClass(), persistentObject.getId());
if(persistentObject instanceof SignalEventSubscriptionEntity) {
createdSignalSubscriptions.remove(persistentObject);
}
}
public EventSubscriptionEntity findEventSubscriptionbyId(String id) {
return (EventSubscriptionEntity) getDbSqlSession().selectOne("selectEventSubscription", id);
}
public long findEventSubscriptionCountByQueryCriteria(EventSubscriptionQueryImpl eventSubscriptionQueryImpl) {
final String query = "selectEventSubscriptionCountByQueryCriteria";
return (Long) getDbSqlSession().selectOne(query, eventSubscriptionQueryImpl);
}
@SuppressWarnings("unchecked")
public List<EventSubscriptionEntity> findEventSubscriptionsByQueryCriteria(EventSubscriptionQueryImpl eventSubscriptionQueryImpl, Page page) {
final String query = "selectEventSubscriptionByQueryCriteria";
return getDbSqlSession().selectList(query, eventSubscriptionQueryImpl, page);
}
@SuppressWarnings("unchecked")
public List<SignalEventSubscriptionEntity> findSignalEventSubscriptionsByEventName(String eventName) {
final String query = "selectSignalEventSubscriptionsByEventName";
Set<SignalEventSubscriptionEntity> selectList = new HashSet<SignalEventSubscriptionEntity>( getDbSqlSession().selectList(query, eventName));
// add events created in this command (not visible yet in query)
for (EventSubscriptionEntity entity : createdSignalSubscriptions) {
if(entity instanceof SignalEventSubscriptionEntity
&& eventName.equals(entity.getEventName())) {
selectList.add((SignalEventSubscriptionEntity) entity);
}
}
return new ArrayList<SignalEventSubscriptionEntity>(selectList);
}
@SuppressWarnings("unchecked")
public List<SignalEventSubscriptionEntity> findSignalEventSubscriptionsByExecution(String executionId) {
final String query = "selectSignalEventSubscriptionsByExecution";
Set<SignalEventSubscriptionEntity> selectList = new HashSet<SignalEventSubscriptionEntity>( getDbSqlSession().selectList(query, executionId));
// add events created in this command (not visible yet in query)
for (EventSubscriptionEntity entity : createdSignalSubscriptions) {
if(entity instanceof SignalEventSubscriptionEntity
&& executionId.equals(entity.getExecutionId())) {
selectList.add((SignalEventSubscriptionEntity) entity);
}
}
return new ArrayList<SignalEventSubscriptionEntity>(selectList);
}
@SuppressWarnings("unchecked")
public List<SignalEventSubscriptionEntity> findSignalEventSubscriptionsByNameAndExecution(String name, String executionId) {
final String query = "selectSignalEventSubscriptionsByNameAndExecution";
Map<String,String> params = new HashMap<String, String>();
params.put("executionId", executionId);
params.put("eventName", name);
Set<SignalEventSubscriptionEntity> selectList = new HashSet<SignalEventSubscriptionEntity>( getDbSqlSession().selectList(query, params));
// add events created in this command (not visible yet in query)
for (EventSubscriptionEntity entity : createdSignalSubscriptions) {
if(entity instanceof SignalEventSubscriptionEntity
&& executionId.equals(entity.getExecutionId())
&& name.equals(entity.getEventName())) {
selectList.add((SignalEventSubscriptionEntity) entity);
}
}
return new ArrayList<SignalEventSubscriptionEntity>(selectList);
}
public List<EventSubscriptionEntity> findEventSubscriptions(String executionId, String type) {
final String query = "selectEventSubscriptionsByExecutionAndType";
Map<String,String> params = new HashMap<String, String>();
params.put("executionId", executionId);
params.put("eventType", type);
return getDbSqlSession().selectList(query, params);
}
public List<EventSubscriptionEntity> findEventSubscriptions(String executionId, String type, String activityId) {
final String query = "selectEventSubscriptionsByExecutionTypeAndActivity";
Map<String,String> params = new HashMap<String, String>();
params.put("executionId", executionId);
params.put("eventType", type);
params.put("activityId", activityId);
return getDbSqlSession().selectList(query, params);
}
public List<EventSubscriptionEntity> findEventSubscriptionsByConfiguration(String type, String configuration) {
final String query = "selectEventSubscriptionsByConfiguration";
Map<String,String> params = new HashMap<String, String>();
params.put("eventType", type);
params.put("configuration", configuration);
return getDbSqlSession().selectList(query, params);
}
public List<EventSubscriptionEntity> findEventSubscriptionByName(String type, String eventName) {
final String query = "selectEventSubscriptionsByName";
Map<String,String> params = new HashMap<String, String>();
params.put("eventType", type);
params.put("eventName", eventName);
return getDbSqlSession().selectList(query, params);
}
public MessageEventSubscriptionEntity findMessageStartEventSubscriptionByName(String messageName) {
MessageEventSubscriptionEntity entity = (MessageEventSubscriptionEntity) getDbSqlSession().selectOne("selectMessageStartEventSubscriptionByName", messageName);
return entity;
}
}
| {'content_hash': 'bd8803cf658c15c4f65c0921afd5f6c4', 'timestamp': '', 'source': 'github', 'line_count': 155, 'max_line_length': 163, 'avg_line_length': 45.42580645161291, 'alnum_prop': 0.7585570231501207, 'repo_name': 'iotsap/FiWare-Template-Handler', 'id': 'a65d31de9099b8a57943bf426750b8eed70496cd', 'size': '7041', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'ExecutionEnvironment/modules/activiti-engine/src/main/java/org/activiti/engine/impl/persistence/entity/EventSubscriptionManager.java', 'mode': '33188', 'license': 'bsd-3-clause', 'language': [{'name': 'CSS', 'bytes': '481305'}, {'name': 'Java', 'bytes': '8276608'}, {'name': 'JavaScript', 'bytes': '3364343'}, {'name': 'Shell', 'bytes': '2529'}, {'name': 'XSLT', 'bytes': '19610'}]} |
"""
Various utilities for working with Python and Matplotlib
"""
import matplotlib
import matplotlib.pyplot as plt
import numpy as np
import os
from math import ceil, sqrt
from skimage.io import imread
def show_images(images,titles=None):
"""Display a list of images"""
n_ims = len(images)
if titles is None: titles = ['(%d)' % i for i in range(1,n_ims + 1)]
fig = plt.figure()
n = 1
for image,title in zip(images, titles):
a = fig.add_subplot(1,n_ims,n) # Make subplot
if image.ndim == 2: # Is image grayscale?
plt.gray() # Only place in this blog you can't replace 'gray' with 'grey'
plt.imshow(image)
a.set_title(title)
n += 1
fig.set_size_inches(np.array(fig.get_size_inches()) * n_ims)
plt.show()
def tile_images(lst, size=None):
"""
Given a list of images, display them in a tiled format.
"""
num_plots = len(lst)
if size is None:
cols = ceil(sqrt(num_plots))
rows = ceil(num_plots/cols)
else:
rows, cols = size
# Set up the figure
fig, axes = plt.subplots(rows, cols)
# Plot the images using `plt.imshow`
for i, x in enumerate(lst):
ax = axes[i]
# If the element is a string, assume that it's a path
if isinstance(x, str):
try:
img = imread(os.path.abspath(x))
ax.imshow(img)
except IOError:
print("Unable to load image: %s" %x)
except Exception as e:
print(e)
# Otherwise, attempt to load it as a numpy array
else:
try:
img = np.array(x)
ax.imshow(img)
except Exception as e:
print(e)
# Show the images
plt.show()
# Return the fig and axes images, in case that is wanted
return fig, axes | {'content_hash': 'd8f79cf4b8fdcc1c30e291cf2f83c6e6', 'timestamp': '', 'source': 'github', 'line_count': 67, 'max_line_length': 85, 'avg_line_length': 28.08955223880597, 'alnum_prop': 0.5626992561105207, 'repo_name': 'clickok/Code-Snippets', 'id': 'f14cf78130b63c45fa8968becbb0cd4d962abf9c', 'size': '1892', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'Python-Programming/utils_pyplot.py', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'C', 'bytes': '2421'}, {'name': 'HTML', 'bytes': '2782'}, {'name': 'JavaScript', 'bytes': '4156'}, {'name': 'Python', 'bytes': '42579'}, {'name': 'Shell', 'bytes': '4041'}]} |
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.contentwarehouse.v1.model;
/**
* Saft named-entities info for a given topic.
*
* <p> This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the contentwarehouse API. For a detailed explanation see:
* <a href="https://developers.google.com/api-client-library/java/google-http-java-client/json">https://developers.google.com/api-client-library/java/google-http-java-client/json</a>
* </p>
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class VideoContentSearchSaftEntityInfo extends com.google.api.client.json.GenericJson {
/**
* Representative canonical name for the entity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String canonicalEntityName;
/**
* Score indicating the saliency (centrality) of this entity to the original_text.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double entitySalience;
/**
* The type name, like "/saft/person", "/saft/art". See README.entity-types for the inventory of
* SAFT type tags.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String entityTypeName;
/**
* Representative entity name mention extracted from original_text.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String mentionText;
/**
* SAFT Mention type.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String mentionType;
/**
* Freebase MID for entity if this the saft entity corresponds to a Webref KG mid. This field is
* not always populated and is taken from FREEBASE_MID mid in EntityProfile in the saft entity
* annotation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String mid;
/**
* The original input text (e.g. the anchor text) where the saft entity annotation was run on.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String originalText;
/**
* Representative canonical name for the entity.
* @return value or {@code null} for none
*/
public java.lang.String getCanonicalEntityName() {
return canonicalEntityName;
}
/**
* Representative canonical name for the entity.
* @param canonicalEntityName canonicalEntityName or {@code null} for none
*/
public VideoContentSearchSaftEntityInfo setCanonicalEntityName(java.lang.String canonicalEntityName) {
this.canonicalEntityName = canonicalEntityName;
return this;
}
/**
* Score indicating the saliency (centrality) of this entity to the original_text.
* @return value or {@code null} for none
*/
public java.lang.Double getEntitySalience() {
return entitySalience;
}
/**
* Score indicating the saliency (centrality) of this entity to the original_text.
* @param entitySalience entitySalience or {@code null} for none
*/
public VideoContentSearchSaftEntityInfo setEntitySalience(java.lang.Double entitySalience) {
this.entitySalience = entitySalience;
return this;
}
/**
* The type name, like "/saft/person", "/saft/art". See README.entity-types for the inventory of
* SAFT type tags.
* @return value or {@code null} for none
*/
public java.lang.String getEntityTypeName() {
return entityTypeName;
}
/**
* The type name, like "/saft/person", "/saft/art". See README.entity-types for the inventory of
* SAFT type tags.
* @param entityTypeName entityTypeName or {@code null} for none
*/
public VideoContentSearchSaftEntityInfo setEntityTypeName(java.lang.String entityTypeName) {
this.entityTypeName = entityTypeName;
return this;
}
/**
* Representative entity name mention extracted from original_text.
* @return value or {@code null} for none
*/
public java.lang.String getMentionText() {
return mentionText;
}
/**
* Representative entity name mention extracted from original_text.
* @param mentionText mentionText or {@code null} for none
*/
public VideoContentSearchSaftEntityInfo setMentionText(java.lang.String mentionText) {
this.mentionText = mentionText;
return this;
}
/**
* SAFT Mention type.
* @return value or {@code null} for none
*/
public java.lang.String getMentionType() {
return mentionType;
}
/**
* SAFT Mention type.
* @param mentionType mentionType or {@code null} for none
*/
public VideoContentSearchSaftEntityInfo setMentionType(java.lang.String mentionType) {
this.mentionType = mentionType;
return this;
}
/**
* Freebase MID for entity if this the saft entity corresponds to a Webref KG mid. This field is
* not always populated and is taken from FREEBASE_MID mid in EntityProfile in the saft entity
* annotation.
* @return value or {@code null} for none
*/
public java.lang.String getMid() {
return mid;
}
/**
* Freebase MID for entity if this the saft entity corresponds to a Webref KG mid. This field is
* not always populated and is taken from FREEBASE_MID mid in EntityProfile in the saft entity
* annotation.
* @param mid mid or {@code null} for none
*/
public VideoContentSearchSaftEntityInfo setMid(java.lang.String mid) {
this.mid = mid;
return this;
}
/**
* The original input text (e.g. the anchor text) where the saft entity annotation was run on.
* @return value or {@code null} for none
*/
public java.lang.String getOriginalText() {
return originalText;
}
/**
* The original input text (e.g. the anchor text) where the saft entity annotation was run on.
* @param originalText originalText or {@code null} for none
*/
public VideoContentSearchSaftEntityInfo setOriginalText(java.lang.String originalText) {
this.originalText = originalText;
return this;
}
@Override
public VideoContentSearchSaftEntityInfo set(String fieldName, Object value) {
return (VideoContentSearchSaftEntityInfo) super.set(fieldName, value);
}
@Override
public VideoContentSearchSaftEntityInfo clone() {
return (VideoContentSearchSaftEntityInfo) super.clone();
}
}
| {'content_hash': '028a514c528c90e5b6c02ef2287de819', 'timestamp': '', 'source': 'github', 'line_count': 219, 'max_line_length': 182, 'avg_line_length': 32.0, 'alnum_prop': 0.7106164383561644, 'repo_name': 'googleapis/google-api-java-client-services', 'id': 'e1b534a626c44015c0a53281ded94c34c5231f87', 'size': '7008', 'binary': False, 'copies': '1', 'ref': 'refs/heads/main', 'path': 'clients/google-api-services-contentwarehouse/v1/2.0.0/com/google/api/services/contentwarehouse/v1/model/VideoContentSearchSaftEntityInfo.java', 'mode': '33188', 'license': 'apache-2.0', 'language': []} |
package org.openspaces.persistency.hibernate.iterator;
import com.gigaspaces.datasource.DataIterator;
import org.hibernate.CacheMode;
import org.hibernate.Criteria;
import org.hibernate.FlushMode;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.Transaction;
import org.hibernate.criterion.Example;
import java.util.Iterator;
/**
* A simple iterator that iterates over template object using Hibernate Criteria
* by example.
*
* @author kimchy
*/
public class DefaultCriteriaByExampleDataIterator implements DataIterator {
protected final Object template;
protected final SessionFactory sessionFactory;
protected Transaction transaction;
protected Session session;
private Iterator iterator;
public DefaultCriteriaByExampleDataIterator(Object template, SessionFactory sessionFactory) {
this.template = template;
this.sessionFactory = sessionFactory;
}
public boolean hasNext() {
if (iterator == null) {
iterator = createIterator();
}
return iterator.hasNext();
}
public Object next() {
return iterator.next();
}
public void remove() {
throw new UnsupportedOperationException("remove not supported");
}
public void close() {
try {
if (transaction == null) {
return;
}
transaction.commit();
} finally {
transaction = null;
if (session != null && session.isOpen()) {
session.close();
}
}
}
protected Iterator createIterator() {
session = sessionFactory.openSession();
transaction = session.beginTransaction();
Example example = Example.create(template);
Criteria criteria = session.createCriteria(template.getClass()).add(example);
criteria.setCacheMode(CacheMode.IGNORE);
criteria.setCacheable(false);
criteria.setFlushMode(FlushMode.NEVER);
return criteria.list().iterator();
}
} | {'content_hash': 'd0c0b7faf564a8a1c8a65d8b6a85774d', 'timestamp': '', 'source': 'github', 'line_count': 78, 'max_line_length': 97, 'avg_line_length': 26.474358974358974, 'alnum_prop': 0.661501210653753, 'repo_name': 'Gigaspaces/xap-openspaces', 'id': 'f4bd31e24e7a4fe52dd66e2fb3e73c192cdb6767', 'size': '2685', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/main/java/org/openspaces/persistency/hibernate/iterator/DefaultCriteriaByExampleDataIterator.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Batchfile', 'bytes': '1107'}, {'name': 'Groovy', 'bytes': '5372'}, {'name': 'HTML', 'bytes': '13501'}, {'name': 'Java', 'bytes': '8666667'}, {'name': 'Shell', 'bytes': '917'}]} |
'use strict';
var gulp = require('gulp'),
gutil = require('gulp-util'),
livereload = require('gulp-livereload'),
notify = require('gulp-notify'),
postcss = require('gulp-postcss'),
rename = require('gulp-rename'),
sass = require('gulp-sass'),
sourcemaps = require('gulp-sourcemaps');
gulp.task('styles', function() {
return gulp.src(['src/scss/screen.scss', 'src/scss/ie.scss'])
.pipe(sourcemaps.init())
.pipe(sass())
.on('error', notify.onError({
icon: 'gulp/developer/icon-notify-styles.jpg',
title: 'SCSS error',
message: '<%= error.message %>'
}))
.pipe(postcss([
require('autoprefixer')({ browsers: [ 'last 3 versions' ]})
]))
.pipe(gutil.env.production ? require('gulp-cssnano')() : gutil.noop())
.pipe(sourcemaps.write('.'))
.pipe(gutil.env.production ? rename(function(path) {
path.basename = path.extname === '.map' ?
path.basename.replace(/\.(.*)$/, '.min.$1') :
path.basename + '.min';
}) : gutil.noop())
.pipe(!gutil.env.production ? livereload() : gutil.noop())
.pipe(gulp.dest('assets/css'))
});
| {'content_hash': '2dd831bba70b52135bac88a619ea3ace', 'timestamp': '', 'source': 'github', 'line_count': 33, 'max_line_length': 72, 'avg_line_length': 35.75757575757576, 'alnum_prop': 0.5703389830508474, 'repo_name': 'dviate/html5-boilerplate', 'id': 'f390374272286db7ad4ae051a881737fd5326fd2', 'size': '1180', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'gulp/tasks/styles.js', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '21549'}, {'name': 'JavaScript', 'bytes': '5758'}, {'name': 'PHP', 'bytes': '2633'}]} |
package com.hazelcast.nio.serialization;
import java.io.IOException;
/**
* Portable provides an alternative serialization method. Instead of relying on reflection, each Portable is
* created by a registered {@link com.hazelcast.nio.serialization.PortableFactory}.
*
* <p>
*
* Portable serialization has the following advantages:
* <ul>
* <li>Support multiple versions of the same object type.
* (See {@link com.hazelcast.config.SerializationConfig#setPortableVersion(int)})</li>
* <li>Fetching individual fields without having to rely on reflection.</li>
* <li>Querying and indexing support without de-serialization and/or reflection.</li>
* </ul>
*
* @see com.hazelcast.nio.serialization.PortableFactory
* @see PortableWriter
* @see PortableReader
* @see ClassDefinition
* @see com.hazelcast.nio.serialization.DataSerializable
* @see com.hazelcast.nio.serialization.IdentifiedDataSerializable
* @see com.hazelcast.config.SerializationConfig
*/
public interface Portable {
/**
* Returns PortableFactory ID for this portable class
* @return factory ID
*/
int getFactoryId();
/**
* Returns class identifier for this portable class. Class ID should be unique per PortableFactory.
* @return class ID
*/
int getClassId();
/**
* Serialize this portable object using PortableWriter
*
* @param writer PortableWriter
* @throws IOException in case of any exceptional case
*/
void writePortable(PortableWriter writer) throws IOException;
/**
* Read portable fields using PortableReader
*
* @param reader PortableReader
* @throws IOException in case of any exceptional case
*/
void readPortable(PortableReader reader) throws IOException;
}
| {'content_hash': 'ef0c58b1203410d3a86cf2a1fe7e4e46', 'timestamp': '', 'source': 'github', 'line_count': 59, 'max_line_length': 108, 'avg_line_length': 30.271186440677965, 'alnum_prop': 0.713885778275476, 'repo_name': 'mdogan/hazelcast', 'id': 'b5beac6b4372050fb4350275bdf72b38351e5f90', 'size': '2411', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'hazelcast/src/main/java/com/hazelcast/nio/serialization/Portable.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Batchfile', 'bytes': '1862'}, {'name': 'C', 'bytes': '3721'}, {'name': 'Java', 'bytes': '45797218'}, {'name': 'Shell', 'bytes': '30002'}]} |
---
uid: SolidEdgePart.Model.ResizeRounds
summary:
remarks:
---
| {'content_hash': 'ce5e33bd1336488ee5160127d24b1e7e', 'timestamp': '', 'source': 'github', 'line_count': 5, 'max_line_length': 37, 'avg_line_length': 13.4, 'alnum_prop': 0.7014925373134329, 'repo_name': 'SolidEdgeCommunity/docs', 'id': '5b3bfdec6a9d597a3b113f327a4f3ee17a551dd6', 'size': '69', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'docfx_project/apidoc/SolidEdgePart.Model.ResizeRounds.md', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Batchfile', 'bytes': '38'}, {'name': 'C#', 'bytes': '5048212'}, {'name': 'C++', 'bytes': '2265'}, {'name': 'CSS', 'bytes': '148'}, {'name': 'PowerShell', 'bytes': '180'}, {'name': 'Smalltalk', 'bytes': '1996'}, {'name': 'Visual Basic', 'bytes': '10236277'}]} |
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<!-- NewPage -->
<html lang="ro">
<head>
<!-- Generated by javadoc (version 1.7.0_07) on Tue May 27 14:37:26 EEST 2014 -->
<title>Uses of Class net.sf.jasperreports.web.util.WebResourceHandlersExtensionRegistryFactory (JasperReports 5.6.0 API)</title>
<meta name="date" content="2014-05-27">
<link rel="stylesheet" type="text/css" href="../../../../../../stylesheet.css" title="Style">
</head>
<body>
<script type="text/javascript"><!--
if (location.href.indexOf('is-external=true') == -1) {
parent.document.title="Uses of Class net.sf.jasperreports.web.util.WebResourceHandlersExtensionRegistryFactory (JasperReports 5.6.0 API)";
}
//-->
</script>
<noscript>
<div>JavaScript is disabled on your browser.</div>
</noscript>
<!-- ========= START OF TOP NAVBAR ======= -->
<div class="topNav"><a name="navbar_top">
<!-- -->
</a><a href="#skip-navbar_top" title="Skip navigation links"></a><a name="navbar_top_firstrow">
<!-- -->
</a>
<ul class="navList" title="Navigation">
<li><a href="../../../../../../overview-summary.html">Overview</a></li>
<li><a href="../package-summary.html">Package</a></li>
<li><a href="../../../../../../net/sf/jasperreports/web/util/WebResourceHandlersExtensionRegistryFactory.html" title="class in net.sf.jasperreports.web.util">Class</a></li>
<li class="navBarCell1Rev">Use</li>
<li><a href="../package-tree.html">Tree</a></li>
<li><a href="../../../../../../deprecated-list.html">Deprecated</a></li>
<li><a href="../../../../../../index-all.html">Index</a></li>
<li><a href="../../../../../../help-doc.html">Help</a></li>
</ul>
</div>
<div class="subNav">
<ul class="navList">
<li>Prev</li>
<li>Next</li>
</ul>
<ul class="navList">
<li><a href="../../../../../../index.html?net/sf/jasperreports/web/util/class-use/WebResourceHandlersExtensionRegistryFactory.html" target="_top">Frames</a></li>
<li><a href="WebResourceHandlersExtensionRegistryFactory.html" target="_top">No Frames</a></li>
</ul>
<ul class="navList" id="allclasses_navbar_top">
<li><a href="../../../../../../allclasses-noframe.html">All Classes</a></li>
</ul>
<div>
<script type="text/javascript"><!--
allClassesLink = document.getElementById("allclasses_navbar_top");
if(window==top) {
allClassesLink.style.display = "block";
}
else {
allClassesLink.style.display = "none";
}
//-->
</script>
</div>
<a name="skip-navbar_top">
<!-- -->
</a></div>
<!-- ========= END OF TOP NAVBAR ========= -->
<div class="header">
<h2 title="Uses of Class net.sf.jasperreports.web.util.WebResourceHandlersExtensionRegistryFactory" class="title">Uses of Class<br>net.sf.jasperreports.web.util.WebResourceHandlersExtensionRegistryFactory</h2>
</div>
<div class="classUseContainer">No usage of net.sf.jasperreports.web.util.WebResourceHandlersExtensionRegistryFactory</div>
<!-- ======= START OF BOTTOM NAVBAR ====== -->
<div class="bottomNav"><a name="navbar_bottom">
<!-- -->
</a><a href="#skip-navbar_bottom" title="Skip navigation links"></a><a name="navbar_bottom_firstrow">
<!-- -->
</a>
<ul class="navList" title="Navigation">
<li><a href="../../../../../../overview-summary.html">Overview</a></li>
<li><a href="../package-summary.html">Package</a></li>
<li><a href="../../../../../../net/sf/jasperreports/web/util/WebResourceHandlersExtensionRegistryFactory.html" title="class in net.sf.jasperreports.web.util">Class</a></li>
<li class="navBarCell1Rev">Use</li>
<li><a href="../package-tree.html">Tree</a></li>
<li><a href="../../../../../../deprecated-list.html">Deprecated</a></li>
<li><a href="../../../../../../index-all.html">Index</a></li>
<li><a href="../../../../../../help-doc.html">Help</a></li>
</ul>
</div>
<div class="subNav">
<ul class="navList">
<li>Prev</li>
<li>Next</li>
</ul>
<ul class="navList">
<li><a href="../../../../../../index.html?net/sf/jasperreports/web/util/class-use/WebResourceHandlersExtensionRegistryFactory.html" target="_top">Frames</a></li>
<li><a href="WebResourceHandlersExtensionRegistryFactory.html" target="_top">No Frames</a></li>
</ul>
<ul class="navList" id="allclasses_navbar_bottom">
<li><a href="../../../../../../allclasses-noframe.html">All Classes</a></li>
</ul>
<div>
<script type="text/javascript"><!--
allClassesLink = document.getElementById("allclasses_navbar_bottom");
if(window==top) {
allClassesLink.style.display = "block";
}
else {
allClassesLink.style.display = "none";
}
//-->
</script>
</div>
<a name="skip-navbar_bottom">
<!-- -->
</a></div>
<!-- ======== END OF BOTTOM NAVBAR ======= -->
<p class="legalCopy"><small>
<span style="font-decoration:none;font-family:Arial,Helvetica,sans-serif;font-size:8pt;font-style:normal;color:#000000;">© 2001-2010 Jaspersoft Corporation <a href="http://www.jaspersoft.com" target="_blank" style="color:#000000;">www.jaspersoft.com</a></span>
</small></p>
</body>
</html>
| {'content_hash': 'df3b3de1364c6959fb007b8cdf824181', 'timestamp': '', 'source': 'github', 'line_count': 120, 'max_line_length': 265, 'avg_line_length': 41.15833333333333, 'alnum_prop': 0.644057501518526, 'repo_name': 'phurtado1112/cnaemvc', 'id': '75d406388f140762dd2f316fca88a274d092ecc7', 'size': '4939', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'lib/JasperReport__5.6/docs/api/net/sf/jasperreports/web/util/class-use/WebResourceHandlersExtensionRegistryFactory.html', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'CSS', 'bytes': '11139'}, {'name': 'HTML', 'bytes': '112926414'}, {'name': 'Java', 'bytes': '532942'}]} |
using System.ComponentModel;
using Windows.ApplicationModel.DataTransfer;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Navigation;
using Windows.UI.Xaml;
using AppStudio.DataProviders.LocalStorage;
using AppStudio.DataProviders.Html;
using NguyenAnNinhConfession.Sections;
using NguyenAnNinhConfession.ViewModels;
using AppStudio.Uwp;
namespace NguyenAnNinhConfession.Pages
{
public sealed partial class AboutThisApp1ListPage : Page
{
public ListViewModel ViewModel { get; set; }
private DataTransferManager _dataTransferManager;
public static readonly DependencyProperty HtmlContentProperty =
DependencyProperty.Register("HtmlContent", typeof(string), typeof(AboutThisApp1ListPage), new PropertyMetadata(string.Empty));
public AboutThisApp1ListPage()
{
this.ViewModel = ListViewModel.CreateNew(Singleton<AboutThisApp1Config>.Instance);
this.InitializeComponent();
}
public string HtmlContent
{
get { return (string)GetValue(HtmlContentProperty); }
set { SetValue(HtmlContentProperty, value); }
}
protected override async void OnNavigatedTo(NavigationEventArgs e)
{
await this.ViewModel.LoadDataAsync();
if (ViewModel.Items != null && ViewModel.Items.Count > 0)
{
HtmlContent = ViewModel.Items[0].Content;
}
_dataTransferManager = DataTransferManager.GetForCurrentView();
_dataTransferManager.DataRequested += OnDataRequested;
base.OnNavigatedTo(e);
}
protected override void OnNavigatedFrom(NavigationEventArgs e)
{
_dataTransferManager.DataRequested -= OnDataRequested;
base.OnNavigatedFrom(e);
}
private void OnDataRequested(DataTransferManager sender, DataRequestedEventArgs args)
{
ViewModel.ShareContent(args.Request);
}
}
}
| {'content_hash': '4867b220652a8fdc8593226508fb6db5', 'timestamp': '', 'source': 'github', 'line_count': 60, 'max_line_length': 138, 'avg_line_length': 32.766666666666666, 'alnum_prop': 0.6912512716174974, 'repo_name': 'goldenskygiang/NANConfessionClient', 'id': 'a37dedffee901d087caa2dd163660e9d180e5407', 'size': '1966', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'UWP/NguyenAnNinhConfession.W10/Pages/AboutThisApp1ListPage.xaml.cs', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'C#', 'bytes': '116846'}, {'name': 'HTML', 'bytes': '157'}]} |
package domain
import (
"encoding/json"
"flag"
"fmt"
"path/filepath"
"testing"
"time"
"go-common/app/interface/main/app-resource/conf"
. "github.com/smartystreets/goconvey/convey"
)
var (
s *Service
)
func WithService(f func(s *Service)) func() {
return func() {
f(s)
}
}
func init() {
dir, _ := filepath.Abs("../../cmd/app-resource-test.toml")
flag.Set("conf", dir)
conf.Init()
s = New(conf.Conf)
time.Sleep(time.Second)
}
func TestDomain(t *testing.T) {
Convey("get Domain data", t, WithService(func(s *Service) {
res := s.Domain()
result, _ := json.Marshal(res)
fmt.Printf("test Domain (%v) \n", string(result))
So(res, ShouldNotBeEmpty)
}))
}
| {'content_hash': '70728a8da29a6801ab7a8ac295963d3c', 'timestamp': '', 'source': 'github', 'line_count': 41, 'max_line_length': 60, 'avg_line_length': 16.634146341463413, 'alnum_prop': 0.6422287390029325, 'repo_name': 'LQJJ/demo', 'id': 'b6971dc274ab1c2054a32c596d786de72ec62ad5', 'size': '682', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': '126-go-common-master/app/interface/main/app-resource/service/domain/domain_test.go', 'mode': '33261', 'license': 'apache-2.0', 'language': [{'name': 'C', 'bytes': '5910716'}, {'name': 'C++', 'bytes': '113072'}, {'name': 'CSS', 'bytes': '10791'}, {'name': 'Dockerfile', 'bytes': '934'}, {'name': 'Go', 'bytes': '40121403'}, {'name': 'Groovy', 'bytes': '347'}, {'name': 'HTML', 'bytes': '359263'}, {'name': 'JavaScript', 'bytes': '545384'}, {'name': 'Makefile', 'bytes': '6671'}, {'name': 'Mathematica', 'bytes': '14565'}, {'name': 'Objective-C', 'bytes': '14900720'}, {'name': 'Objective-C++', 'bytes': '20070'}, {'name': 'PureBasic', 'bytes': '4152'}, {'name': 'Python', 'bytes': '4490569'}, {'name': 'Ruby', 'bytes': '44850'}, {'name': 'Shell', 'bytes': '33251'}, {'name': 'Swift', 'bytes': '463286'}, {'name': 'TSQL', 'bytes': '108861'}]} |
<?php
/**
* vpopqmail
*
* @license http://opensource.org/licenses/MIT MIT
*/
interface MazelabVpopqmail_Model_Dataprovider_Interface_MailingList
{
/**
* deletes a certain mailing list
*
* @param MazelabVpopqmail_Model_ValueObject_MailingList $mailingList
* @return boolean
*/
public function deleteMailingList(MazelabVpopqmail_Model_ValueObject_MailingList $mailingList);
/**
* returns content of mailing list from given id
*
* @param string $id
* @return array
*/
public function getMailingList($id);
/**
* returns content of mailing list from given email
*
* @param string $email
* @return array
*/
public function getMailingListByEmail($email);
/**
* get all mailing lists
*
* @return array
*/
public function getMailingLists();
/**
* returns all mailing lists of a certain domain
*
* @param string $id
* @return array
*/
public function getMailingListsByDomain($domainId);
/**
* saves given data set to a certain mailing list
*
* if id is not provided, it will create a new item
*
* @param string $mailingListId
* @param array $data
* @return string|false id of saved item
*/
public function saveMailingList(array $data, $mailingListId = null);
}
| {'content_hash': '18419dbe5561a568092fbc102c4dfbff', 'timestamp': '', 'source': 'github', 'line_count': 61, 'max_line_length': 99, 'avg_line_length': 22.918032786885245, 'alnum_prop': 0.6137339055793991, 'repo_name': 'mazelab/vpopqmail', 'id': '36385a76c1743fab192cb17615d118ed67a40c95', 'size': '1398', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'models/Dataprovider/Interface/MailingList.php', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '4074'}, {'name': 'PHP', 'bytes': '486295'}]} |
#ifndef ALINOUS_RUNTIME_PARALLEL_CONCURRENTTASKEXEXUTOR_H_
#define ALINOUS_RUNTIME_PARALLEL_CONCURRENTTASKEXEXUTOR_H_
namespace alinous{namespace annotation{
class OneSource;
}}
namespace alinous {namespace runtime {namespace parallel {
class ThreadPool;}}}
namespace alinous {namespace runtime {namespace parallel {
class LaunchJoin;}}}
namespace alinous {namespace runtime {namespace parallel {
class IParallelTask;}}}
namespace alinous {namespace runtime {namespace parallel {
class ConcurrentTaskQueue;}}}
namespace java {namespace lang {
class InterruptedException;}}
namespace java {namespace util {
template <typename T> class LinkedList;}}
namespace alinous {namespace lock {
class LockObject;}}
namespace alinous {namespace runtime {namespace parallel {
class AlinousThread;}}}
namespace alinous {namespace runtime {namespace parallel {
class ConcurrentTaskExexutor;}}}
namespace alinous {namespace runtime {namespace parallel {
class IThreadAction;}}}
namespace java {namespace lang {
class IObject;
}}
namespace alinous {
class ThreadContext;
}
namespace alinous {namespace runtime {namespace parallel {
using namespace ::alinous;
using namespace ::java::lang;
using ::java::util::Iterator;
using ::java::util::LinkedList;
using ::alinous::lock::LockObject;
class ConcurrentTaskExexutor final : public virtual IObject {
public:
class FinalyzerEntryPoint;
class LauncherEntryPoint;
ConcurrentTaskExexutor(const ConcurrentTaskExexutor& base) = default;
public:
ConcurrentTaskExexutor(ThreadPool* pool, ThreadContext* ctx) throw() ;
void __construct_impl(ThreadPool* pool, ThreadContext* ctx) throw() ;
virtual ~ConcurrentTaskExexutor() throw();
virtual void __releaseRegerences(bool prepare, ThreadContext* ctx) throw();
private:
LinkedList<ConcurrentTaskQueue>* queue;
LinkedList<ConcurrentTaskQueue>* readyQueue;
LockObject* sync;
AlinousThread* finalizer;
LaunchJoin* finalizerLaunchJoin;
AlinousThread* launcher;
LaunchJoin* launcherLaunchJoin;
bool finalizerEnds;
ConcurrentTaskQueue* lastTask;
ThreadPool* pool;
public:
void begin(ThreadContext* ctx);
void startTask(IParallelTask* task, ThreadContext* ctx) throw() ;
void flush(ThreadContext* ctx);
void dispose(ThreadContext* ctx) throw() ;
private:
void finalizeLoop(ThreadContext* ctx);
void launcherLoop(ThreadContext* ctx);
void asyncStart(ThreadContext* ctx);
public:
class FinalyzerEntryPoint final : public IThreadAction, public virtual IObject {
public:
FinalyzerEntryPoint(const FinalyzerEntryPoint& base) = default;
public:
FinalyzerEntryPoint(ConcurrentTaskExexutor* exec, ThreadContext* ctx) throw() ;
void __construct_impl(ConcurrentTaskExexutor* exec, ThreadContext* ctx) throw() ;
virtual ~FinalyzerEntryPoint() throw();
virtual void __releaseRegerences(bool prepare, ThreadContext* ctx) throw();
private:
ConcurrentTaskExexutor* executor;
public:
void execute(ThreadContext* ctx) throw() final;
public:
static bool __init_done;
static bool __init_static_variables();
public:
static void __cleanUp(ThreadContext* ctx);
};
class LauncherEntryPoint final : public IThreadAction, public virtual IObject {
public:
LauncherEntryPoint(const LauncherEntryPoint& base) = default;
public:
LauncherEntryPoint(ConcurrentTaskExexutor* exec, ThreadContext* ctx) throw() ;
void __construct_impl(ConcurrentTaskExexutor* exec, ThreadContext* ctx) throw() ;
virtual ~LauncherEntryPoint() throw();
virtual void __releaseRegerences(bool prepare, ThreadContext* ctx) throw();
private:
ConcurrentTaskExexutor* exec;
public:
void execute(ThreadContext* ctx) throw() final;
public:
static bool __init_done;
static bool __init_static_variables();
public:
static void __cleanUp(ThreadContext* ctx);
};
static bool __init_done;
static bool __init_static_variables();
public:
static void __cleanUp(ThreadContext* ctx);
};
}}}
#endif /* end of ALINOUS_RUNTIME_PARALLEL_CONCURRENTTASKEXEXUTOR_H_ */
| {'content_hash': '3f6d1978433b3314e4955bc3b3804649', 'timestamp': '', 'source': 'github', 'line_count': 137, 'max_line_length': 83, 'avg_line_length': 28.934306569343065, 'alnum_prop': 0.7754793138244198, 'repo_name': 'alinous-core/alinous-elastic-db', 'id': '375ae14ae348efc22ccb8918a484917129be7022', 'size': '3964', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'lib/src_java/alinous.runtime.parallel/ConcurrentTaskExexutor.h', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'C', 'bytes': '7447'}, {'name': 'C++', 'bytes': '14486500'}, {'name': 'CMake', 'bytes': '223064'}]} |
layout: post
title: Insertion sort on small arrays in merge sort
date: 2015-08-30 15:04
tags: CLRS Algorithms
---
2.1 Although merge sort runs in $$\Theta(n \lg n)$$ worst-case time and isnertion sort runs in $$\Theta(n^2)$$ worst-case time, the constant factors in insertion sort can make it faster in practice for small problem sizes on many machines. Thus, it makes sense to coarsen the leaves of the recursion by using insertion sort within merge sort when subproblems become sufficiently small. Consider a modification to merge sort in which $$n/k$$ sublists of length $$k$$ are sorted using insertion sort and then merged using the standard merging mechanism, where $$k$$ is a value to be determined.
1. Show that insertion sort can sort the $$n/k$$ sublists, each of length $$k$$, in $$\Theta(nk)$$ worst-case time.
We know that insertion sort runs in $$\Theta(n^2)$$ time where $$n$$ is the size of the list. Thus when the list is of size $$k$$, the worst case time is $$\Theta(k^2)$$. Repeating this $$n/k$$ times (once for each of the $$n/k$$ sublists), we get $$n/k\Theta(k^2)=\Theta(nk)$$.
2. Show how to merge the sublists in $$\Theta(n\lg(n/k))$$ worst-case time.
We merge sublists the exact same way as we did in regular mergesort. As before, there are $$n$$ elements at each level. Thus the merges cost $$\Theta(n)$$ at each level. To see how many levels there are we note that recursion bottoms out when the size of the list is less than $$k$$, thus the number of levels, $$l$$, satisfies $$n(\frac{1}{2}^l)\leq k \Rightarrow n\leq k2^l \Rightarrow n/k \leq 2^l \Rightarrow \lg(n/k) \leq l$$. Therefore $$l = \lceil\lg(n/k)\rceil$$ and we can merge sublists in $$\Theta(n\lg(n/k))$$ worst-case time.
3. Given that the modified algorithm runs in $$\Theta(nk + n\lg(n/k))$$ worst-case time, what is the largest value of $$k$$ as a function of $$n$$ for which the modified algorithm has the same running time as standard merge sort, in terms of $$\Theta$$ notation?
Merge sort runs in $$\Theta(n\lg n)$$ time. Thus for the modified algorithm to have a asymptotically faster run time, $$\Theta(nk + n\lg(n/k)) < \Theta(n\lg n) \Rightarrow max(nk, n\lg(n/k)) < n\lg n$$, we must have $$nk < n\lg n$$ and $$n\lg(n/k) < n\lg n$$. Therefore, $$1 < k < \lg n$$.
As $$nk$$ strictly increases with $$k$$ and $$n\lg(n/k)$$ strictly decreases with $$k$$, the lowest asymptotic running time occurs when $$k$$ is such that $$nk = n\lg(n/k)$$.
{% highlight python %}
def merge(left, right):
''' as before this function merges two lists, producing a new one '''
sorted_keys = list()
while True:
try:
left_top = left[0]
right_top = right[0]
except IndexError:
sorted_keys += left + right
return sorted_keys
if left_top < right_top:
sorted_keys.append(left.pop(0))
else:
sorted_keys.append(right.pop(0))
def insertion_sort(keys):
''' in place insertion sort '''
for i in xrange(1,len(keys)):
value = keys[i]
j = i - 1
while j >= 0 and keys[j] > value:
keys[j + 1] = keys[j]
j -= 1
keys[j + 1] = value
def modified_merge_sort(keys, k):
if len(keys) <= k:
insertion_sort(keys)
return keys
else:
midpoint = len(keys) / 2
left = modified_merge_sort(keys[:midpoint], k)
right = modified_merge_sort(keys[midpoint:], k)
return merge(left, right)
{% endhighlight %}
| {'content_hash': '3537999a0a5a9dae0d8466a0caa43ddd', 'timestamp': '', 'source': 'github', 'line_count': 60, 'max_line_length': 592, 'avg_line_length': 58.56666666666667, 'alnum_prop': 0.6442800227660785, 'repo_name': 'alw231/alw231.github.io', 'id': 'c4cadf8d2a016a792fb46e79b0069ab5a7831160', 'size': '3518', 'binary': False, 'copies': '1', 'ref': 'refs/heads/disable_gh_pages', 'path': '_posts/2015-08-30-insertion-sort-on-small-arrays-in-merge-sort.md', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'CSS', 'bytes': '9664'}, {'name': 'HTML', 'bytes': '13345'}, {'name': 'JavaScript', 'bytes': '27423'}]} |
/* ########## PARA VISUALIZAR Y REGISTRAR PUBLICACIONES ########## */
/*Estilo en el título (centrar el texto)*/
.title-style{
text-align: center;
}
/*Color para icono de borrado en autores*/
.btn-delete-color{
color: #d9534f;
}
/*Espaciado superior e inferior en mensaje de alerta (no hay resultados de autor)*/
.spacing-text-alert{
margin-top: 10px;
margin-bottom: 10px;
}
/*Margen, espaciado y color para el formulario "Registrar autor"*/
.subform-autor{
background-color: #dedede;
padding: 15px;
margin-bottom: 1em;
}
/*Decoración de fondo en cada año*/
.blockquote-decoration{
border-left: 5px solid #666;
background-color: #ddd;
}
/*Espacio para mostrar publicación con imagen
Se emplean publication-container, publication-cover y publication-info*/
.publication-container{ /*contenedor principal*/
height: 305px;
width: 100%;
margin-bottom: 20px;
position: relative; /*para alinear texto posteriormente*/
}
.publication-cover{ /*espacio para portada*/
height: 100%;
width: 100%;
overflow: hidden;
display: flex;
justify-content: center;
align-items: center;
background-color: #333;
}
.publication-cover img{
width: 100%;
}
.publication-info{ /*espacio para texto*/
height: 30%;
width: 100%;
position: absolute;
bottom: 0;
font-size: 1.2em;
text-align: center;
background-color: rgba(0,0,0,0.5);
color: #eee;
}
/*Definir tamaños, espacios y alineación de cada recuardo.
También se define el tamaño individual del título, autor, fecha*/
.publication-thumbnail{
height: 305px;
width: 100%;
padding: 1em;
margin: auto;
margin-bottom: 20px;
text-align: center;
}
.publication-title{
font-size: 1.3em;
font-weight: bold;
}
.publication-autor{
font-size: 1em;
}
.publication-date{
font-size: 1.2em;
}
/*Color de fondo y de texto si la publicación es un ARTICULO*/
.color-article{
background: #133175;
background: -webkit-linear-gradient(#133175, #0E4671);
background: -o-linear-gradient(#133175, #0E4671);
background: -moz-linear-gradient(#133175, #0E4671);
background: linear-gradient(#133175, #0E4671);
}
.color-article-title{
color: #F0A32F;
}
.color-article-autor{
color: #FFF;
}
.color-article-date{
color: #BBB;
}
/*Color de fondo y de texto si la publicación es un LIBRO*/
.color-book{
background: #19197B;
background: -webkit-linear-gradient(#19197B, #132F76);
background: -o-linear-gradient(#19197B, #132F76);
background: -moz-linear-gradient(#19197B, #132F76);
background: linear-gradient(#19197B, #132F76);
}
.color-book-title{
color: #F0A32F;
}
.color-book-autor{
color: #FFF;
}
.color-book-date{
color: #BBB;
}
/*Color de fondo y de texto si la publicación es un DOCUMENTAL*/
.color-documentary{
background: #0E4671;
background: -webkit-linear-gradient(#0E4671, #07666A);
background: -o-linear-gradient(#0E4671, #07666A);
background: -moz-linear-gradient(#0E4671, #07666A);
background: linear-gradient(#0E4671, #07666A);
}
.color-documentary-title{
color: #F0A32F;
}
.color-documentary-autor{
color: #FFF;
}
.color-documentary-date{
color: #BBB;
}
/*Color de fondo y de texto si la publicación es una PONENCIA*/
.color-lecture{
background: #2C1479;
background: -webkit-linear-gradient(#2C1479, #1B197A);
background: -o-linear-gradient(#2C1479, #1B197A);
background: -moz-linear-gradient(#2C1479, #1B197A);
background: linear-gradient(#2C1479, #1B197A);
}
.color-lecture-title{
color: #F0A32F;
}
.color-lecture-autor{
color: #FFF;
}
.color-lecture-date{
color: #BBB;
}
/*Color de fondo y de texto si la publicación es una PAGINA WEB*/
.color-website{
background: #076969;
background: -webkit-linear-gradient(#076969, #07774A);
background: -o-linear-gradient(#076969, #07774A);
background: -moz-linear-gradient(#076969, #07774A);
background: linear-gradient(#076969, #07774A);
}
.color-website-title{
color: #F0A32F;
}
.color-website-autor{
color: #FFF;
}
.color-website-date{
color: #BBB;
}
/*Color de fondo y de texto si la publicación no tiene un tipo definido*/
.color-default{
background: #666;
background: -webkit-linear-gradient(#666, #999);
background: -o-linear-gradient(#666, #999);
background: -moz-linear-gradient(#666, #999);
background: linear-gradient(#666, #999);
}
.color-default-title{
color: #EEE;
}
.color-default-autor{
color: #FFF;
}
.color-default-date{
color: #BBB;
}
/*Cada una de las propiedades de la publicación (en tamaño xs)*/
.row-property{
margin-bottom: 0.5em;
margin-top: 0.5em;
padding-top: 0.5em;
border-top-style: solid;
border-top-color: rgba(0,0,0,0.1);
border-top-width: 1px;
}
/*Imagen responsiva (max-width: 100% está en estilo de <img>)*/
.imagen-portada{
max-height: 400px;
}
/*Estilo a usar en tamaño sm, md, lg*/
@media screen and (min-width: 768px){
/*Se disminuye ligeramente el tamaño de fuente*/
.publication-title{
font-size: 1.2em;
}
.publication-autor{
font-size: 0.9em;
}
.publication-date{
font-size: 1em;
}
} | {'content_hash': '498455a51bb0c83f40e7e5f9136b1abc', 'timestamp': '', 'source': 'github', 'line_count': 219, 'max_line_length': 83, 'avg_line_length': 22.365296803652967, 'alnum_prop': 0.708248264597795, 'repo_name': 'Sergioarc/lais-web', 'id': '5bdfeac2fc20bc045ffcb47bf86d7aa8eaa758ab', 'size': '4918', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'public/css/publicaciones.css', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '18104'}, {'name': 'HTML', 'bytes': '105358'}, {'name': 'JavaScript', 'bytes': '73154'}]} |
package net.systran.platform.resources.client.model;
import net.systran.platform.resources.client.model.LiteCorpus;
import io.swagger.annotations.*;
import com.fasterxml.jackson.annotation.JsonProperty;
@ApiModel(description = "")
public class CorpusImportResponse {
private LiteCorpus corpus = null;
/**
**/
@ApiModelProperty(required = true, value = "")
@JsonProperty("corpus")
public LiteCorpus getCorpus() {
return corpus;
}
public void setCorpus(LiteCorpus corpus) {
this.corpus = corpus;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CorpusImportResponse {\n");
sb.append(" corpus: ").append(corpus).append("\n");
sb.append("}\n");
return sb.toString();
}
}
| {'content_hash': 'c66ec28f456289e2f2d6e9c6a62add87', 'timestamp': '', 'source': 'github', 'line_count': 39, 'max_line_length': 62, 'avg_line_length': 20.333333333333332, 'alnum_prop': 0.6796973518284993, 'repo_name': 'SYSTRAN/resources-api-java-client', 'id': '2ff574e8be00fdc9a7337d45012cf1b0d5954a1c', 'size': '1420', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/main/java/net/systran/platform/resources/client/model/CorpusImportResponse.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Java', 'bytes': '293321'}]} |
<?php
namespace Drupal\commerce\Element;
use Drupal\Component\Utility\NestedArray;
use Drupal\Core\Form\FormStateInterface;
use Drupal\Core\Render\Element;
/**
* Provides a trait for Commerce form elements.
*
* Allows form elements to use #commerce_element_submit, a substitute
* for the #element_submit that's missing from Drupal core.
*
* Each form element using this trait should add the attachElementSubmit and
* validateElementSubmit callbacks to their getInfo() methods.
*/
trait CommerceElementTrait {
/**
* Attaches the #commerce_element_submit functionality.
*
* @param array $element
* The form element being processed.
* @param \Drupal\Core\Form\FormStateInterface $form_state
* The current state of the form.
* @param array $complete_form
* The complete form structure.
*
* @return array
* The processed form element.
*/
public static function attachElementSubmit(array $element, FormStateInterface $form_state, array &$complete_form) {
if (isset($complete_form['#commerce_element_submit_attached'])) {
return $element;
}
// The #validate callbacks of the complete form run last.
// That allows executeElementSubmitHandlers() to be completely certain that
// the form has passed validation before proceeding.
$complete_form['#validate'][] = [get_class(), 'executeElementSubmitHandlers'];
$complete_form['#commerce_element_submit_attached'] = TRUE;
return $element;
}
/**
* Confirms that #commerce_element_submit handlers can be run.
*
* @param array $element
* The form element.
* @param \Drupal\Core\Form\FormStateInterface $form_state
* The current state of the form.
*
* @throws \Exception
* Thrown if button-level #validate handlers are detected on the parent
* form, as a protection against buggy behavior.
*/
public static function validateElementSubmit(array &$element, FormStateInterface $form_state) {
// Button-level #validate handlers replace the form-level ones, which means
// that executeElementSubmitHandlers() won't be triggered.
if ($handlers = $form_state->getValidateHandlers()) {
throw new \Exception('The current form must not have button-level #validate handlers');
}
}
/**
* Submits elements by calling their #commerce_element_submit callbacks.
*
* Form API has no #element_submit, requiring us to simulate it by running
* the #commerce_element_submit handlers either in the last step of
* validation, or the first step of submission. In this case it's the last
* step of validation, allowing thrown exceptions to be converted into form
* errors.
*
* @param array &$form
* The form.
* @param \Drupal\Core\Form\FormStateInterface $form_state
* The form state.
*/
public static function executeElementSubmitHandlers(array &$form, FormStateInterface $form_state) {
if (!$form_state->isSubmitted() || $form_state->hasAnyErrors()) {
// The form wasn't submitted (#ajax in progress) or failed validation.
return;
}
// A submit button might need to process only a part of the form.
// For example, the "Apply coupon" button at checkout should apply coupons,
// but not save the payment information. Use #limit_validation_errors
// as a guideline for which parts of the form to submit.
$triggering_element = $form_state->getTriggeringElement();
if (isset($triggering_element['#limit_validation_errors']) && $triggering_element['#limit_validation_errors'] !== FALSE) {
// #limit_validation_errors => [], the button cares about nothing.
if (empty($triggering_element['#limit_validation_errors'])) {
return;
}
foreach ($triggering_element['#limit_validation_errors'] as $limit_validation_errors) {
$element = NestedArray::getValue($form, $limit_validation_errors);
if (!$element) {
// The element will be empty if #parents don't match #array_parents,
// the case for IEF widgets. In that case just submit everything.
$element = &$form;
}
self::doExecuteSubmitHandlers($element, $form_state);
}
}
else {
self::doExecuteSubmitHandlers($form, $form_state);
}
}
/**
* Calls the #commerce_element_submit callbacks recursively.
*
* @param array &$element
* The current element.
* @param \Drupal\Core\Form\FormStateInterface $form_state
* The form state.
*/
public static function doExecuteSubmitHandlers(array &$element, FormStateInterface $form_state) {
// Recurse through all children.
foreach (Element::children($element) as $key) {
if (!empty($element[$key])) {
static::doExecuteSubmitHandlers($element[$key], $form_state);
}
}
// If there are callbacks on this level, run them.
if (!empty($element['#commerce_element_submit'])) {
foreach ($element['#commerce_element_submit'] as $callback) {
call_user_func_array($callback, [&$element, &$form_state]);
}
}
}
}
| {'content_hash': 'f22376fbca3314e460f55723563035c5', 'timestamp': '', 'source': 'github', 'line_count': 135, 'max_line_length': 126, 'avg_line_length': 37.51851851851852, 'alnum_prop': 0.6797630799605133, 'repo_name': 'wow-yorick/suffix-zx', 'id': 'abf844fa916ea5597f5029f68bd5f6a960c271d9', 'size': '5065', 'binary': False, 'copies': '5', 'ref': 'refs/heads/master', 'path': 'cms/modules/contrib/commerce/src/Element/CommerceElementTrait.php', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '711070'}, {'name': 'HTML', 'bytes': '944057'}, {'name': 'JavaScript', 'bytes': '1530798'}, {'name': 'PHP', 'bytes': '39866961'}, {'name': 'Shell', 'bytes': '55615'}]} |
package com.google.devtools.j2objc;
import com.google.devtools.j2objc.util.ErrorUtil;
import com.google.devtools.j2objc.util.JdtParser;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.lang.reflect.Constructor;
import java.net.MalformedURLException;
import java.net.URL;
import java.net.URLClassLoader;
import java.util.Arrays;
import java.util.jar.JarEntry;
import java.util.jar.JarInputStream;
import java.util.logging.Level;
import java.util.logging.Logger;
/**
* Translation tool for generating Objective C source files from Java sources.
* This tool is not intended to be a general purpose converter, but instead is
* focused on what is needed for business logic libraries written in Java to
* run natively on iOS. In particular, no attempt is made to translate Java
* UI framework code to any iOS frameworks.
*
* @author Tom Ball
*/
public class J2ObjC {
static {
// Always enable assertions in translator.
ClassLoader loader = J2ObjC.class.getClassLoader();
if (loader != null) {
loader.setPackageAssertionStatus(J2ObjC.class.getPackage().getName(), true);
}
}
private static final Logger logger = Logger.getLogger(J2ObjC.class.getName());
private static void exit() {
Options.deleteTemporaryDirectory();
System.exit(ErrorUtil.errorCount());
}
public static String getFileHeader(String sourceFileName) {
return String.format(Options.getFileHeader(), sourceFileName);
}
private static class JarFileLoader extends URLClassLoader {
public JarFileLoader() {
super(new URL[]{});
}
public void addJarFile(String path) throws MalformedURLException {
String urlPath = "jar:file://" + path + "!/";
addURL(new URL(urlPath));
}
}
private static void initPlugins(String[] pluginPaths, String pluginOptionString)
throws IOException {
@SuppressWarnings("resource")
JarFileLoader classLoader = new JarFileLoader();
for (String path : pluginPaths) {
if (path.endsWith(".jar")) {
JarInputStream jarStream = null;
try {
jarStream = new JarInputStream(new FileInputStream(path));
classLoader.addJarFile(new File(path).getAbsolutePath());
JarEntry entry;
while ((entry = jarStream.getNextJarEntry()) != null) {
String entryName = entry.getName();
if (!entryName.endsWith(".class")) {
continue;
}
String className = entryName.replaceAll("/", "\\.").substring(
0, entryName.length() - ".class".length());
try {
Class<?> clazz = classLoader.loadClass(className);
if (Plugin.class.isAssignableFrom(clazz)) {
Constructor<?> cons = clazz.getDeclaredConstructor();
Plugin plugin = (Plugin) cons.newInstance();
plugin.initPlugin(pluginOptionString);
Options.getPlugins().add(plugin);
}
} catch (Exception e) {
throw new IOException("plugin exception: ", e);
}
}
} finally {
if (jarStream != null) {
jarStream.close();
}
}
} else {
logger.warning("Don't understand plugin path entry: " + path);
}
}
}
public static void error(Exception e) {
logger.log(Level.SEVERE, "Exiting due to exception", e);
System.exit(1);
}
private static void checkErrors() {
int errors = ErrorUtil.errorCount();
if (Options.treatWarningsAsErrors()) {
errors += ErrorUtil.warningCount();
}
if (errors > 0) {
System.exit(1);
}
}
private static JdtParser createParser() {
JdtParser parser = new JdtParser();
parser.addClasspathEntries(Options.getClassPathEntries());
parser.addClasspathEntries(Options.getBootClasspath());
parser.addSourcepathEntries(Options.getSourcePathEntries());
parser.setIncludeRunningVMBootclasspath(false);
parser.setEncoding(Options.fileEncoding());
parser.setIgnoreMissingImports(Options.ignoreMissingImports());
parser.setEnableDocComments(Options.docCommentsEnabled());
return parser;
}
/**
* Entry point for tool.
*
* @param args command-line arguments: flags and source file names
* @throws IOException
*/
public static void main(String[] args) {
if (args.length == 0) {
Options.help(true);
}
String[] files = null;
try {
files = Options.load(args);
if (files.length == 0) {
Options.usage("no source files");
}
} catch (IOException e) {
ErrorUtil.error(e.getMessage());
System.exit(1);
}
try {
initPlugins(Options.getPluginPathEntries(), Options.getPluginOptionString());
} catch (IOException e) {
error(e);
}
JdtParser parser = createParser();
// Remove dead-code first, so modified file paths are replaced in the
// translation list.
DeadCodeProcessor deadCodeProcessor = DeadCodeProcessor.create(parser);
if (deadCodeProcessor != null) {
deadCodeProcessor.processFiles(Arrays.asList(files));
checkErrors();
files = deadCodeProcessor.postProcess().toArray(new String[0]);
}
TranslationProcessor translationProcessor = new TranslationProcessor(parser);
translationProcessor.processFiles(Arrays.asList(files));
translationProcessor.postProcess();
checkErrors();
exit();
}
}
| {'content_hash': '76e911b5678ecc4ec70a92e643a024c1', 'timestamp': '', 'source': 'github', 'line_count': 178, 'max_line_length': 83, 'avg_line_length': 30.803370786516854, 'alnum_prop': 0.6567572496808317, 'repo_name': 'xuvw/j2objc', 'id': 'eeae7c59084b2f2297c37d87366743668a09de83', 'size': '6094', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'translator/src/main/java/com/google/devtools/j2objc/J2ObjC.java', 'mode': '33188', 'license': 'apache-2.0', 'language': []} |
//------------------------------------------------------------------------------
// <auto-generated>
// This code was generated by a tool.
// Runtime Version:4.0.30319.1
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
// </auto-generated>
//------------------------------------------------------------------------------
namespace ScrimpNet.Presentation.Demo.Properties {
[global::System.Runtime.CompilerServices.CompilerGeneratedAttribute()]
[global::System.CodeDom.Compiler.GeneratedCodeAttribute("Microsoft.VisualStudio.Editors.SettingsDesigner.SettingsSingleFileGenerator", "10.0.0.0")]
internal sealed partial class Settings : global::System.Configuration.ApplicationSettingsBase {
private static Settings defaultInstance = ((Settings)(global::System.Configuration.ApplicationSettingsBase.Synchronized(new Settings())));
public static Settings Default {
get {
return defaultInstance;
}
}
}
}
| {'content_hash': '1015b4f54b3019450c30737be8c6b284', 'timestamp': '', 'source': 'github', 'line_count': 26, 'max_line_length': 151, 'avg_line_length': 41.46153846153846, 'alnum_prop': 0.5844155844155844, 'repo_name': 'lindexi/lindexi_gd', 'id': '0ab21252e442d1c49617ae4f0c1ad5f5e23bc262', 'size': '1080', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'ScrimpNet.Library.Suite Solution/__SamplesAndDemos/Presentation/ScrimpNet.Presentation.Demo Project/Properties/Settings.Designer.cs', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'ASP.NET', 'bytes': '29822'}, {'name': 'Batchfile', 'bytes': '57394'}, {'name': 'C', 'bytes': '983450'}, {'name': 'C#', 'bytes': '36237467'}, {'name': 'C++', 'bytes': '316762'}, {'name': 'CSS', 'bytes': '121116'}, {'name': 'Dockerfile', 'bytes': '882'}, {'name': 'HTML', 'bytes': '113044'}, {'name': 'JavaScript', 'bytes': '3323'}, {'name': 'Q#', 'bytes': '556'}, {'name': 'Roff', 'bytes': '450615'}, {'name': 'ShaderLab', 'bytes': '3270'}, {'name': 'Smalltalk', 'bytes': '18'}, {'name': 'TSQL', 'bytes': '23558'}]} |
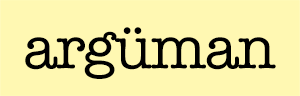
----
[Arguman.org](http://arguman.org) is an argument analysis and [mapping](https://en.wikipedia.org/wiki/Argument_map) platform.
## How it works
Users assert contentions to be discussed, supported, prooved or disprooved and argue with premises using because, but, or however conjuctions.

## Benefits
Critical thinking is the intellectually disciplined process of actively and skillfully conceptualizing, applying, analyzing, synthesizing, and/or evaluating information gathered from, or generated by, observation, experience, reflection, reasoning, or communication, as a guide to belief and action. In its exemplary form, it is based on universal intellectual values that transcend subject matter divisions: clarity, accuracy, precision, consistency, relevance, sound evidence, good reasons, depth, breadth, and fairness.
Basis of critical thinking is arguments. Assesment is done over argument, argument premises, promotives and corruptives.
You may think argument mappings as visual hierarchy mappings.
Arguman.org’s aim is arguments to be mapped successfully by many users.

## Who are we
arguman.org is an open source project which developed by community, If you want to contribute technically or intellectually please don’t hesitate.
### Contributors
- Fatih Erikli
- Tuna Vargı
- Huseyin Mert
- Erdem Ozkol
- Aybars Badur
- Can Göktaş
- Bahattin Çiniç
- Murat Çorlu
- Cumhur Korkut
- Serdar Dalgıç
- Emir Karşıyakalı
- Ali Barın
- Halil Kaya
- A. Liva Cengiz
- Çağlar Bozkurt
## Additionally Thanks To
- Burak Arıkan
- Kadir Akkara Yardımcı
| {'content_hash': '26dfd58d440f166f9d99a533bb9c2256', 'timestamp': '', 'source': 'github', 'line_count': 50, 'max_line_length': 522, 'avg_line_length': 36.5, 'alnum_prop': 0.7857534246575343, 'repo_name': 'taiansu/arguman.org', 'id': '3a784adce01562b8f7e84172ff227bb29a4e6de4', 'size': '1847', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'README.md', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '43835'}, {'name': 'HTML', 'bytes': '57145'}, {'name': 'JavaScript', 'bytes': '23644'}, {'name': 'Python', 'bytes': '163449'}, {'name': 'Shell', 'bytes': '392'}]} |
#ifndef RMLUI_CORE_LAYOUTLINEBOX_H
#define RMLUI_CORE_LAYOUTLINEBOX_H
#include "LayoutInlineBox.h"
namespace Rml {
class LayoutBlockBox;
/**
@author Peter Curry
*/
class LayoutLineBox
{
public:
LayoutLineBox(LayoutBlockBox* parent);
~LayoutLineBox();
/// Closes the line box, positioning all inline elements within it.
/// @param overflow[in] The overflow box from a split inline box that caused this line to close. Leave this as nullptr if we closed naturally.
/// @return If there was any overflow, this will be the last element generated by the spilling content. Otherwise, this will be nullptr.
LayoutInlineBox* Close(UniquePtr<LayoutInlineBox> overflow = nullptr);
/// Closes one of the line box's inline boxes.
/// @param inline_box[in] The inline box to close. This should always be the line box's open box.
void CloseInlineBox(LayoutInlineBox* inline_box);
/// Attempts to add a new element to this line box. If it can't fit, or needs to be split, new line boxes will
/// be created. The inline box for the final section of the element will be returned.
/// @param element[in] The element to fit into this line box.
/// @param box[in] The element's extents.
/// @return The inline box for the element.
LayoutInlineBox* AddElement(Element* element, const Box& box);
/// Attempts to add a new inline box to this line. If it can't fit, or needs to be split, new line boxes will
/// be created. The inline box for the final section of the element will be returned.
/// @param box[in] The inline box to be added to the line.
/// @return The final inline box.
LayoutInlineBox* AddBox(UniquePtr<LayoutInlineBox> box);
/// Adds an inline box as a chained hierarchy overflowing to this line. The chain will be extended into
/// this line box.
/// @param split_box[in] The box overflowed from a previous line.
void AddChainedBox(LayoutInlineBox* chained_box);
/// Returns the position of the line box, relative to its parent's block box's content area.
/// @return The position of the line box.
Vector2f GetPosition() const;
/// Returns the position of the line box, relative to its parent's block box's offset parent.
/// @return The relative position of the line box.
Vector2f GetRelativePosition() const;
/// Returns the dimensions of the line box.
/// @return The dimensions of the line box.
Vector2f GetDimensions() const;
/// Returns the line box's open inline box.
/// @return The line's open inline box, or nullptr if it currently has none.
LayoutInlineBox* GetOpenInlineBox();
/// Returns the line's containing block box.
/// @return The line's block box.
LayoutBlockBox* GetBlockBox();
float GetBoxCursor() const;
bool GetBaselineOfLastLine(float& baseline) const;
void* operator new(size_t size);
void operator delete(void* chunk, size_t size);
private:
/// Appends an inline box to the end of the line box's list of inline boxes. Returns a pointer to the appended box.
LayoutInlineBox* AppendBox(UniquePtr<LayoutInlineBox> box);
using InlineBoxList = Vector< UniquePtr<LayoutInlineBox> >;
// The block box containing this line.
LayoutBlockBox* parent;
// The top-left position of the line box; this is set when the first inline box is placed.
Vector2f position;
bool position_set;
// The width and height of the line box; this is set when the line box is placed.
Vector2f dimensions;
bool wrap_content;
// The horizontal cursor. This is where the next inline box will be placed along the line.
float box_cursor;
// The list of inline boxes in this line box. These line boxes may be parented to others in this list.
InlineBoxList inline_boxes;
// The open inline box; this is nullptr if all inline boxes are closed.
LayoutInlineBox* open_inline_box;
};
} // namespace Rml
#endif
| {'content_hash': '3f16b37514b0fb5e7df448d883c007d4', 'timestamp': '', 'source': 'github', 'line_count': 99, 'max_line_length': 143, 'avg_line_length': 38.121212121212125, 'alnum_prop': 0.7419183889772125, 'repo_name': 'rokups/Urho3D', 'id': '7a3de761f03fa87c7cea22d76b35e79dc84f2e45', 'size': '5122', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'Source/ThirdParty/RmlUi/Source/Core/LayoutLineBox.h', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Batchfile', 'bytes': '14842'}, {'name': 'C#', 'bytes': '643494'}, {'name': 'C++', 'bytes': '7624116'}, {'name': 'CMake', 'bytes': '285041'}, {'name': 'GLSL', 'bytes': '151027'}, {'name': 'HLSL', 'bytes': '175276'}, {'name': 'HTML', 'bytes': '23451'}, {'name': 'Java', 'bytes': '89032'}, {'name': 'MAXScript', 'bytes': '94704'}, {'name': 'Makefile', 'bytes': '1161'}, {'name': 'Objective-C', 'bytes': '6549'}, {'name': 'Python', 'bytes': '31194'}, {'name': 'Shell', 'bytes': '27209'}]} |
package me.tikitoo.androiddemo.activity;
import android.app.Activity;
import android.content.Context;
import android.content.Intent;
import android.net.Uri;
import android.os.Build;
import android.os.Bundle;
import android.os.Environment;
import android.support.annotation.Nullable;
import android.view.View;
import android.widget.Toast;
import java.io.File;
import java.io.FileOutputStream;
import java.io.OutputStream;
import me.tikitoo.androiddemo.R;
import me.tikitoo.androiddemo.view.DrawView;
/**
* Created by Tikitoo on 2015/12/22.
*/
public class DrawViewActivity extends Activity {
private DrawView mDrawView;
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_custom_view);
mDrawView = (DrawView) findViewById(R.id.draw_view);
}
public void saveBtn(View view) {
onSaveClicked(this, view);
}
public void onSaveClicked(final Context context, View view) {
try {
File file = new File(Environment.getExternalStorageDirectory(),
System.currentTimeMillis() + ".jpg");
OutputStream outputStream = new FileOutputStream(file);
mDrawView.saveBitmap(outputStream);
outputStream.close();
/*
Intent intent = new Intent();
intent.setAction(Intent.ACTION_MEDIA_MOUNTED);
intent.setData(Uri.fromFile(Environment.getExternalStorageDirectory()));
sendBroadcast(intent);*/
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.KITKAT) {
Intent mediaScanIntent = new Intent(
Intent.ACTION_MEDIA_SCANNER_SCAN_FILE);
Uri contentUri = Uri.fromFile(file);
mediaScanIntent.setData(contentUri);
this.sendBroadcast(mediaScanIntent);
} else {
sendBroadcast(new Intent(
Intent.ACTION_MEDIA_MOUNTED,
Uri.fromFile(Environment.getExternalStorageDirectory())));
}
showToast(context, "save success");
} catch (Exception e) {
showToast(context, "save failed");
e.printStackTrace();
}
}
private static void showToast(Context context, String msg) {
Toast.makeText(context, msg, Toast.LENGTH_SHORT).show();
}
}
| {'content_hash': 'b7142dc4152dfaa5c8a26c0381091fe6', 'timestamp': '', 'source': 'github', 'line_count': 77, 'max_line_length': 84, 'avg_line_length': 31.532467532467532, 'alnum_prop': 0.6437397034596376, 'repo_name': 'Tikitoo/androidsamples', 'id': 'cdf574b03eb9ca4612851c2ba6f7ec1438750a8e', 'size': '2428', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'app/src/main/java/me/tikitoo/androiddemo/activity/DrawViewActivity.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'HTML', 'bytes': '1744'}, {'name': 'Java', 'bytes': '128274'}]} |
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<!-- NewPage -->
<html lang="en">
<head>
<!-- Generated by javadoc -->
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Uses of Class com.google.zxing.client.j2se.GUIRunner (ZXing 3.3.0 API)</title>
<link rel="stylesheet" type="text/css" href="../../../../../../stylesheet.css" title="Style">
<script type="text/javascript" src="../../../../../../script.js"></script>
</head>
<body>
<script type="text/javascript"><!--
try {
if (location.href.indexOf('is-external=true') == -1) {
parent.document.title="Uses of Class com.google.zxing.client.j2se.GUIRunner (ZXing 3.3.0 API)";
}
}
catch(err) {
}
//-->
</script>
<noscript>
<div>JavaScript is disabled on your browser.</div>
</noscript>
<!-- ========= START OF TOP NAVBAR ======= -->
<div class="topNav"><a name="navbar.top">
<!-- -->
</a>
<div class="skipNav"><a href="#skip.navbar.top" title="Skip navigation links">Skip navigation links</a></div>
<a name="navbar.top.firstrow">
<!-- -->
</a>
<ul class="navList" title="Navigation">
<li><a href="../../../../../../overview-summary.html">Overview</a></li>
<li><a href="../package-summary.html">Package</a></li>
<li><a href="../../../../../../com/google/zxing/client/j2se/GUIRunner.html" title="class in com.google.zxing.client.j2se">Class</a></li>
<li class="navBarCell1Rev">Use</li>
<li><a href="../package-tree.html">Tree</a></li>
<li><a href="../../../../../../deprecated-list.html">Deprecated</a></li>
<li><a href="../../../../../../index-all.html">Index</a></li>
<li><a href="../../../../../../help-doc.html">Help</a></li>
</ul>
</div>
<div class="subNav">
<ul class="navList">
<li>Prev</li>
<li>Next</li>
</ul>
<ul class="navList">
<li><a href="../../../../../../index.html?com/google/zxing/client/j2se/class-use/GUIRunner.html" target="_top">Frames</a></li>
<li><a href="GUIRunner.html" target="_top">No Frames</a></li>
</ul>
<ul class="navList" id="allclasses_navbar_top">
<li><a href="../../../../../../allclasses-noframe.html">All Classes</a></li>
</ul>
<div>
<script type="text/javascript"><!--
allClassesLink = document.getElementById("allclasses_navbar_top");
if(window==top) {
allClassesLink.style.display = "block";
}
else {
allClassesLink.style.display = "none";
}
//-->
</script>
</div>
<a name="skip.navbar.top">
<!-- -->
</a></div>
<!-- ========= END OF TOP NAVBAR ========= -->
<div class="header">
<h2 title="Uses of Class com.google.zxing.client.j2se.GUIRunner" class="title">Uses of Class<br>com.google.zxing.client.j2se.GUIRunner</h2>
</div>
<div class="classUseContainer">No usage of com.google.zxing.client.j2se.GUIRunner</div>
<!-- ======= START OF BOTTOM NAVBAR ====== -->
<div class="bottomNav"><a name="navbar.bottom">
<!-- -->
</a>
<div class="skipNav"><a href="#skip.navbar.bottom" title="Skip navigation links">Skip navigation links</a></div>
<a name="navbar.bottom.firstrow">
<!-- -->
</a>
<ul class="navList" title="Navigation">
<li><a href="../../../../../../overview-summary.html">Overview</a></li>
<li><a href="../package-summary.html">Package</a></li>
<li><a href="../../../../../../com/google/zxing/client/j2se/GUIRunner.html" title="class in com.google.zxing.client.j2se">Class</a></li>
<li class="navBarCell1Rev">Use</li>
<li><a href="../package-tree.html">Tree</a></li>
<li><a href="../../../../../../deprecated-list.html">Deprecated</a></li>
<li><a href="../../../../../../index-all.html">Index</a></li>
<li><a href="../../../../../../help-doc.html">Help</a></li>
</ul>
</div>
<div class="subNav">
<ul class="navList">
<li>Prev</li>
<li>Next</li>
</ul>
<ul class="navList">
<li><a href="../../../../../../index.html?com/google/zxing/client/j2se/class-use/GUIRunner.html" target="_top">Frames</a></li>
<li><a href="GUIRunner.html" target="_top">No Frames</a></li>
</ul>
<ul class="navList" id="allclasses_navbar_bottom">
<li><a href="../../../../../../allclasses-noframe.html">All Classes</a></li>
</ul>
<div>
<script type="text/javascript"><!--
allClassesLink = document.getElementById("allclasses_navbar_bottom");
if(window==top) {
allClassesLink.style.display = "block";
}
else {
allClassesLink.style.display = "none";
}
//-->
</script>
</div>
<a name="skip.navbar.bottom">
<!-- -->
</a></div>
<!-- ======== END OF BOTTOM NAVBAR ======= -->
<p class="legalCopy"><small>Copyright © 2007–2016. All rights reserved.</small></p>
</body>
</html>
| {'content_hash': 'a631a97e448ba4906443a1ae44504b42', 'timestamp': '', 'source': 'github', 'line_count': 125, 'max_line_length': 139, 'avg_line_length': 36.344, 'alnum_prop': 0.6048866387849439, 'repo_name': 'DavidLDawes/zxing', 'id': 'd964903a8c27f11a3a690fe43baeb808a0084c0a', 'size': '4543', 'binary': False, 'copies': '3', 'ref': 'refs/heads/master', 'path': 'docs/apidocs/com/google/zxing/client/j2se/class-use/GUIRunner.html', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'CSS', 'bytes': '3869'}, {'name': 'HTML', 'bytes': '102287'}, {'name': 'Java', 'bytes': '2367385'}]} |
FROM balenalib/orangepi-plus2-debian:bullseye-run
RUN apt-get update \
&& apt-get install -y --no-install-recommends \
ca-certificates \
curl \
\
# .NET Core dependencies
libc6 \
libgcc1 \
libgssapi-krb5-2 \
libicu67 \
libssl1.1 \
libstdc++6 \
zlib1g \
&& rm -rf /var/lib/apt/lists/*
# Configure web servers to bind to port 80 when present
ENV ASPNETCORE_URLS=http://+:80 \
# Enable detection of running in a container
DOTNET_RUNNING_IN_CONTAINER=true
# Install .NET Core
ENV DOTNET_VERSION 3.1.21
RUN curl -SL --output dotnet.tar.gz "https://dotnetcli.blob.core.windows.net/dotnet/Runtime/$DOTNET_VERSION/dotnet-runtime-$DOTNET_VERSION-linux-arm.tar.gz" \
&& dotnet_sha512='9c3fb0f5f860f53ab4d15124c2c23a83412ea916ad6155c0f39f066057dcbb3ca6911ae26daf8a36dbfbc09c17d6565c425fbdf3db9114a28c66944382b71000' \
&& echo "$dotnet_sha512 dotnet.tar.gz" | sha512sum -c - \
&& mkdir -p /usr/share/dotnet \
&& tar -zxf dotnet.tar.gz -C /usr/share/dotnet \
&& rm dotnet.tar.gz \
&& ln -s /usr/share/dotnet/dotnet /usr/bin/dotnet
CMD ["echo","'No CMD command was set in Dockerfile! Details about CMD command could be found in Dockerfile Guide section in our Docs. Here's the link: https://balena.io/docs"]
RUN curl -SLO "https://raw.githubusercontent.com/balena-io-library/base-images/44e597e40f2010cdde15b3ba1e397aea3a5c5271/scripts/assets/tests/[email protected]" \
&& echo "Running test-stack@dotnet" \
&& chmod +x [email protected] \
&& bash [email protected] \
&& rm -rf [email protected]
RUN [ ! -d /.balena/messages ] && mkdir -p /.balena/messages; echo 'Here are a few details about this Docker image (For more information please visit https://www.balena.io/docs/reference/base-images/base-images/): \nArchitecture: ARM v7 \nOS: Debian Bullseye \nVariant: run variant \nDefault variable(s): UDEV=off \nThe following software stack is preinstalled: \ndotnet 3.1-runtime \nExtra features: \n- Easy way to install packages with `install_packages <package-name>` command \n- Run anywhere with cross-build feature (for ARM only) \n- Keep the container idling with `balena-idle` command \n- Show base image details with `balena-info` command' > /.balena/messages/image-info
RUN echo '#!/bin/sh.real\nbalena-info\nrm -f /bin/sh\ncp /bin/sh.real /bin/sh\n/bin/sh "$@"' > /bin/sh-shim \
&& chmod +x /bin/sh-shim \
&& cp /bin/sh /bin/sh.real \
&& mv /bin/sh-shim /bin/sh | {'content_hash': 'c9a576a3bf9b47b40d470a5ebb785172', 'timestamp': '', 'source': 'github', 'line_count': 47, 'max_line_length': 682, 'avg_line_length': 53.42553191489362, 'alnum_prop': 0.702110712863401, 'repo_name': 'resin-io-library/base-images', 'id': 'e6a7f214a12bc4e397eabf388386351029627da1', 'size': '2532', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'balena-base-images/dotnet/orangepi-plus2/debian/bullseye/3.1-runtime/run/Dockerfile', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Dockerfile', 'bytes': '71234697'}, {'name': 'JavaScript', 'bytes': '13096'}, {'name': 'Shell', 'bytes': '12051936'}, {'name': 'Smarty', 'bytes': '59789'}]} |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Code Coverage for /var/www/em/module/Em/src/Em/Controller/IndexController.php</title>
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link href="css/bootstrap.min.css" rel="stylesheet">
<link href="css/bootstrap-responsive.min.css" rel="stylesheet">
<link href="css/style.css" rel="stylesheet">
<!--[if lt IE 9]>
<script src="http://html5shim.googlecode.com/svn/trunk/html5.js"></script>
<![endif]-->
</head>
<body>
<header>
<div class="container">
<div class="row">
<div class="span12">
<ul class="breadcrumb">
<li><a href="index.html">/var/www/em/module</a> <span class="divider">/</span></li>
<li><a href="Em.html">Em</a> <span class="divider">/</span></li>
<li><a href="Em_src.html">src</a> <span class="divider">/</span></li>
<li><a href="Em_src_Em.html">Em</a> <span class="divider">/</span></li>
<li><a href="Em_src_Em_Controller.html">Controller</a> <span class="divider">/</span></li>
<li class="active">IndexController.php</li>
</ul>
</div>
</div>
</div>
</header>
<div class="container">
<table class="table table-bordered">
<thead>
<tr>
<td> </td>
<td colspan="10"><div align="center"><strong>Code Coverage</strong></div></td>
</tr>
<tr>
<td> </td>
<td colspan="3"><div align="center"><strong>Classes and Traits</strong></div></td>
<td colspan="4"><div align="center"><strong>Functions and Methods</strong></div></td>
<td colspan="3"><div align="center"><strong>Lines</strong></div></td>
</tr>
</thead>
<tbody>
<tr>
<td class="danger">Total</td>
<td class="danger big"> <div class="progress progress-danger" style="width: 100px;">
<div class="bar" style="width: 0.00%;"></div>
</div>
</td>
<td class="danger small"><div align="right">0.00%</div></td>
<td class="danger small"><div align="right">0 / 1</div></td>
<td class="danger big"> <div class="progress progress-danger" style="width: 100px;">
<div class="bar" style="width: 0.00%;"></div>
</div>
</td>
<td class="danger small"><div align="right">0.00%</div></td>
<td class="danger small"><div align="right">0 / 1</div></td>
<td class="danger small"><acronym title="Change Risk Anti-Patterns (CRAP) Index">CRAP</acronym></td>
<td class="danger big"> <div class="progress progress-danger" style="width: 100px;">
<div class="bar" style="width: 0.00%;"></div>
</div>
</td>
<td class="danger small"><div align="right">0.00%</div></td>
<td class="danger small"><div align="right">0 / 4</div></td>
</tr>
<tr>
<td class="danger">IndexController</td>
<td class="danger big"> <div class="progress progress-danger" style="width: 100px;">
<div class="bar" style="width: 0.00%;"></div>
</div>
</td>
<td class="danger small"><div align="right">0.00%</div></td>
<td class="danger small"><div align="right">0 / 1</div></td>
<td class="danger big"> <div class="progress progress-danger" style="width: 100px;">
<div class="bar" style="width: 0.00%;"></div>
</div>
</td>
<td class="danger small"><div align="right">0.00%</div></td>
<td class="danger small"><div align="right">0 / 1</div></td>
<td class="danger small">2</td>
<td class="danger big"> <div class="progress progress-danger" style="width: 100px;">
<div class="bar" style="width: 0.00%;"></div>
</div>
</td>
<td class="danger small"><div align="right">0.00%</div></td>
<td class="danger small"><div align="right">0 / 4</div></td>
</tr>
<tr>
<td class="danger" colspan="4"> <a href="#17">indexAction()</a></td>
<td class="danger big"> <div class="progress progress-danger" style="width: 100px;">
<div class="bar" style="width: 0.00%;"></div>
</div>
</td>
<td class="danger small"><div align="right">0.00%</div></td>
<td class="danger small"><div align="right">0 / 1</div></td>
<td class="danger small">2</td>
<td class="danger big"> <div class="progress progress-danger" style="width: 100px;">
<div class="bar" style="width: 0.00%;"></div>
</div>
</td>
<td class="danger small"><div align="right">0.00%</div></td>
<td class="danger small"><div align="right">0 / 4</div></td>
</tr>
</tbody>
</table>
<table class="table table-borderless table-condensed">
<tbody>
<tr><td><div align="right"><a name="1"></a><a href="#1">1</a></div></td><td class="codeLine"><span class="default"><?php</span></td></tr>
<tr><td><div align="right"><a name="2"></a><a href="#2">2</a></div></td><td class="codeLine"><span class="comment"></span></td></tr>
<tr><td><div align="right"><a name="9"></a><a href="#9">9</a></div></td><td class="codeLine"></td></tr>
<tr><td><div align="right"><a name="10"></a><a href="#10">10</a></div></td><td class="codeLine"><span class="keyword">namespace</span><span class="default"> </span><span class="default">Em</span><span class="default">\</span><span class="default">Controller</span><span class="keyword">;</span></td></tr>
<tr><td><div align="right"><a name="11"></a><a href="#11">11</a></div></td><td class="codeLine"></td></tr>
<tr><td><div align="right"><a name="12"></a><a href="#12">12</a></div></td><td class="codeLine"><span class="keyword">use</span><span class="default"> </span><span class="default">Zend</span><span class="default">\</span><span class="default">Mvc</span><span class="default">\</span><span class="default">Controller</span><span class="default">\</span><span class="default">AbstractActionController</span><span class="keyword">;</span></td></tr>
<tr><td><div align="right"><a name="13"></a><a href="#13">13</a></div></td><td class="codeLine"><span class="keyword">use</span><span class="default"> </span><span class="default">Zend</span><span class="default">\</span><span class="default">View</span><span class="default">\</span><span class="default">Model</span><span class="default">\</span><span class="default">ViewModel</span><span class="keyword">;</span></td></tr>
<tr><td><div align="right"><a name="14"></a><a href="#14">14</a></div></td><td class="codeLine"></td></tr>
<tr><td><div align="right"><a name="15"></a><a href="#15">15</a></div></td><td class="codeLine"><span class="keyword">class</span><span class="default"> </span><span class="default">IndexController</span><span class="default"> </span><span class="keyword">extends</span><span class="default"> </span><span class="default">AbstractActionController</span></td></tr>
<tr><td><div align="right"><a name="16"></a><a href="#16">16</a></div></td><td class="codeLine"><span class="keyword">{</span></td></tr>
<tr class="danger"><td><div align="right"><a name="17"></a><a href="#17">17</a></div></td><td class="codeLine"><span class="default"> </span><span class="keyword">public</span><span class="default"> </span><span class="keyword">function</span><span class="default"> </span><span class="default">indexAction</span><span class="keyword">(</span><span class="keyword">)</span></td></tr>
<tr class="danger"><td><div align="right"><a name="18"></a><a href="#18">18</a></div></td><td class="codeLine"><span class="default"> </span><span class="keyword">{</span></td></tr>
<tr class="danger"><td><div align="right"><a name="19"></a><a href="#19">19</a></div></td><td class="codeLine"><span class="default"> </span><span class="keyword">return</span><span class="default"> </span><span class="keyword">new</span><span class="default"> </span><span class="default">ViewModel</span><span class="keyword">(</span><span class="keyword">)</span><span class="keyword">;</span></td></tr>
<tr class="danger"><td><div align="right"><a name="20"></a><a href="#20">20</a></div></td><td class="codeLine"><span class="default"> </span><span class="keyword">}</span></td></tr>
<tr><td><div align="right"><a name="21"></a><a href="#21">21</a></div></td><td class="codeLine"><span class="keyword">}</span></td></tr>
</tbody>
</table>
<footer>
<h4>Legend</h4>
<p>
<span class="success"><strong>Executed</strong></span>
<span class="danger"><strong>Not Executed</strong></span>
<span class="warning"><strong>Dead Code</strong></span>
</p>
<p>
<small>Generated by <a href="http://github.com/sebastianbergmann/php-code-coverage" target="_top">PHP_CodeCoverage 1.2.6</a> using <a href="http://www.php.net/" target="_top">PHP 5.4.12-2~precise+1</a> and <a href="http://phpunit.de/">PHPUnit 3.7.8</a> at Tue Apr 16 12:43:11 CEST 2013.</small>
</p>
</footer>
</div>
<script src="js/jquery.min.js" type="text/javascript"></script>
<script src="js/bootstrap.min.js" type="text/javascript"></script>
<script type="text/javascript">$('.popin').popover({trigger: 'hover'});</script>
</body>
</html>
| {'content_hash': '4b9113c0451c2e6a06009ccdcba45c41', 'timestamp': '', 'source': 'github', 'line_count': 149, 'max_line_length': 468, 'avg_line_length': 62.375838926174495, 'alnum_prop': 0.5993113836884011, 'repo_name': 'kpurrmann/em', 'id': '701d7ed44d27da6dcdad05959d7ca2b7b864bb80', 'size': '10623', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'module/Event/test/log/Em_src_Em_Controller_IndexController.php.html', 'mode': '33188', 'license': 'bsd-3-clause', 'language': [{'name': 'JavaScript', 'bytes': '41651'}, {'name': 'PHP', 'bytes': '99453'}]} |
package at.ac.tuwien.dsg.mela.common.monitoringConcepts;
import java.io.Serializable;
import javax.xml.bind.annotation.*;
/**
* Author: Daniel Moldovan E-Mail: [email protected] *
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlRootElement(name = "Action")
public class Action implements Serializable {
@XmlAttribute(name = "targetEntityID", required = true)
private String targetEntityID;
@XmlAttribute(name = "action", required = true)
private String action;
public Action() {
}
public Action(String targetEntityID, String action) {
this.targetEntityID = targetEntityID;
this.action = action;
}
public String getTargetEntityID() {
return targetEntityID;
}
public void setTargetEntityID(String targetEntityID) {
this.targetEntityID = targetEntityID;
}
public String getAction() {
return action;
}
public void setAction(String action) {
this.action = action;
}
@Override
public int hashCode() {
int hash = 7;
hash = 31 * hash + (this.targetEntityID != null ? this.targetEntityID.hashCode() : 0);
hash = 31 * hash + (this.action != null ? this.action.hashCode() : 0);
return hash;
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (getClass() != obj.getClass()) {
return false;
}
final Action other = (Action) obj;
if ((this.targetEntityID == null) ? (other.targetEntityID != null) : !this.targetEntityID.equals(other.targetEntityID)) {
return false;
}
if ((this.action == null) ? (other.action != null) : !this.action.equals(other.action)) {
return false;
}
return true;
}
public Action withTargetEntityID(final String targetEntityID) {
this.targetEntityID = targetEntityID;
return this;
}
public Action withAction(final String action) {
this.action = action;
return this;
}
public Action clone() {
return new Action(targetEntityID, action);
}
}
| {'content_hash': '3f7a8789baa4184800054608c7525a08', 'timestamp': '', 'source': 'github', 'line_count': 86, 'max_line_length': 129, 'avg_line_length': 25.348837209302324, 'alnum_prop': 0.6096330275229358, 'repo_name': 'tuwiendsg/MELA', 'id': 'e41ea791e2108e16d2f5113bd1e1a73a04800728', 'size': '2958', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'MELA-Core/MELA-Common/src/main/java/at/ac/tuwien/dsg/mela/common/monitoringConcepts/Action.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'CSS', 'bytes': '245937'}, {'name': 'HTML', 'bytes': '844879'}, {'name': 'Java', 'bytes': '2859855'}, {'name': 'JavaScript', 'bytes': '1208998'}, {'name': 'Python', 'bytes': '203971'}, {'name': 'R', 'bytes': '12601'}, {'name': 'Shell', 'bytes': '1906'}]} |
using System;
using System.Collections.Generic;
using System.Data.Entity.ModelConfiguration;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Dominio.Entidades;
namespace Infraestrutura.Configuracao.EF
{
public class TipoTelefoneConfiguration
:EntityTypeConfiguration<TipoTelefone>
{
}
}
| {'content_hash': '59e557ef1c37fb4e8e7bcee10aa38989', 'timestamp': '', 'source': 'github', 'line_count': 15, 'max_line_length': 46, 'avg_line_length': 22.333333333333332, 'alnum_prop': 0.7850746268656716, 'repo_name': 'gabrielsimas/SistemasExemplo', 'id': '39d71fb46c1ca88e078a70be73445d22cda8c07a', 'size': '337', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'DDD/ControleContatos/Infraestrutura/Configuracao/EF/TipoTelefoneConfiguration.cs', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'ASP', 'bytes': '14994'}, {'name': 'C#', 'bytes': '456492'}, {'name': 'CSS', 'bytes': '2056'}, {'name': 'HTML', 'bytes': '14829'}, {'name': 'Java', 'bytes': '13901'}, {'name': 'JavaScript', 'bytes': '322489'}]} |
var getPixels = require('canvas-pixels').get3d;
var SpriteBatch = require('./');
var test = require('tape').test;
test('draws texture colors', function(t) {
var gl = require('webgl-context')({ width: 1, height: 1 });
//a white texture is often useful for tinting lines and rectangles
var tex = require('kami-white-texture')(gl);
var batch = new SpriteBatch(gl);
batch.begin();
batch.setColor(1, 0, 0, 1);
batch.draw(tex, 0, 0, 10, 10);
batch.end();
var pix = getPixels(gl);
t.ok( pix[0]===255 && pix[1]===0 && pix[2]===0, 'setColor tints texture color' );
t.end();
}); | {'content_hash': '917601c26c8f144c5c85d80a9f3af9b9', 'timestamp': '', 'source': 'github', 'line_count': 22, 'max_line_length': 85, 'avg_line_length': 28.272727272727273, 'alnum_prop': 0.6028938906752411, 'repo_name': 'mattdesl/kami-batch', 'id': 'f3e6a39dc4aa5f7db7817177464443d0f320dc05', 'size': '622', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'test.js', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'JavaScript', 'bytes': '13958'}]} |
<!DOCTYPE html>
<html>
<head>
<title>Posts Tagged “Lesson” – devStory – Tales from a web developer</title>
<meta charset="utf-8" />
<meta content='text/html; charset=utf-8' http-equiv='Content-Type'>
<meta http-equiv='X-UA-Compatible' content='IE=edge'>
<meta name="twitter:card" content="summary_large_image">
<meta name="twitter:image" content="http://www.devstory.mikecornish.net/images/social-large.png">
<meta property="og:image" content="http://www.devstory.mikecornish.net/images/social-large.png">
<meta name='viewport' content='width=device-width, initial-scale=1.0, maximum-scale=1.0'>
<meta name="twitter:site" content="@MikeWCornish">
<meta name="twitter:creator" content="@MikeWCornish">
<meta name="description" content="Mike Cornish is a compulsive creator who builds websites.">
<meta property="og:description" content="Mike Cornish is a compulsive creator who builds websites." />
<meta name="twitter:description" content="Mike Cornish is a compulsive creator who builds websites." />
<meta name="author" content="devStory" />
<meta property="og:title" content="" />
<meta property="twitter:title" content="" />
<!--[if lt IE 9]>
<script src="http://html5shiv.googlecode.com/svn/trunk/html5.js"></script>
<![endif]-->
<link rel="stylesheet" type="text/css" href="/style.css" />
<link rel="alternate" type="application/rss+xml" title="devStory - Tales from a web developer" href="/feed.xml" />
<link href='https://fonts.googleapis.com/css?family=Merriweather:400,300,700|Merriweather+Sans:700,400italic,400' rel='stylesheet' type='text/css'>
<!-- Created with Jekyll Now - http://github.com/barryclark/jekyll-now -->
</head>
<body>
<div class="wrapper-masthead">
<div class="container">
<header class="masthead clearfix">
<!--<a href="/" class="site-avatar"><img src="https://raw.githubusercontent.com/barryclark/jekyll-now/master/images/jekyll-logo.png" /></a>-->
<div class="site-info">
<h1 class="site-name"><a class="logo" href="/" data-logo>devStory</a>
<script type="text/javascript" src="/js/logo.js"></script>
<script type="text/javascript">
initLogo();
</script>
</h1>
<p class="site-description">Tales from a web developer</p>
</div>
<nav>
<a class="about" href="/about">mikeCornish</a>
</nav>
</header>
</div>
</div>
<div id="main" role="main" class="container">
<h2 class="post_title">Posts Tagged “Lesson”</h2>
<ul>
<li class="archive_list">
<time style="color:#666;font-size:11px;" datetime='2017-03-07'>03/07/17</time> <a class="archive_list_article_link" href='/posts/chapter-7/'>#FailMore</a>
<p class="summary"></p>
<ul class="tag_list">
<li class="inline archive_list"><a class="tag_list_link" href="/tag/Chapter">Chapter</a></li>
<li class="inline archive_list"><a class="tag_list_link" href="/tag/Life">Life</a></li>
<li class="inline archive_list"><a class="tag_list_link" href="/tag/Lesson">Lesson</a></li>
</ul>
</li>
</ul>
</div>
<div class="wrapper-footer">
<div class="container">
<footer class="footer">
<a href="mailto:[email protected]" target="_blank"><i class="svg-icon email"></i></a>
<a href="https://github.com/mCornish" target="_blank"><i class="svg-icon github"></i></a>
<a href="https://www.twitter.com/MikeWCornish"><i class="svg-icon twitter"></i></a>
</footer>
</div>
</div>
<!-- start Mixpanel -->
<script type="text/javascript">
(function(e,b){if(!b.__SV){var a,f,i,g;window.mixpanel=b;b._i=[];b.init=function(a,e,d){function f(b,h){var a=h.split(".");2==a.length&&(b=b[a[0]],h=a[1]);b[h]=function(){b.push([h].concat(Array.prototype.slice.call(arguments,0)))}}var c=b;"undefined"!==typeof d?c=b[d]=[]:d="mixpanel";c.people=c.people||[];c.toString=function(b){var a="mixpanel";"mixpanel"!==d&&(a+="."+d);b||(a+=" (stub)");return a};c.people.toString=function(){return c.toString(1)+".people (stub)"};i="disable time_event track track_pageview track_links track_forms register register_once alias unregister identify name_tag set_config people.set people.set_once people.increment people.append people.union people.track_charge people.clear_charges people.delete_user".split(" ");
for(g=0;g<i.length;g++)f(c,i[g]);b._i.push([a,e,d])};b.__SV=1.2;a=e.createElement("script");a.type="text/javascript";a.async=!0;a.src="undefined"!==typeof MIXPANEL_CUSTOM_LIB_URL?MIXPANEL_CUSTOM_LIB_URL:"file:"===e.location.protocol&&"//cdn.mxpnl.com/libs/mixpanel-2-latest.min.js".match(/^\/\//)?"https://cdn.mxpnl.com/libs/mixpanel-2-latest.min.js":"//cdn.mxpnl.com/libs/mixpanel-2-latest.min.js";f=e.getElementsByTagName("script")[0];f.parentNode.insertBefore(a,f)}})(document,window.mixpanel||[]);
mixpanel.init("6da672b6f66f9813757d30f733fa3758");
</script>
<!-- end Mixpanel -->
<script src="/js/analytics.js" type="text/javascript"></script>
<script type="text/javascript">
trackPageview();
trackLinks();
</script>
</body>
</html>
| {'content_hash': '1920bc47ffd7530bc75ba2412a04ea15', 'timestamp': '', 'source': 'github', 'line_count': 215, 'max_line_length': 750, 'avg_line_length': 25.823255813953487, 'alnum_prop': 0.6080691642651297, 'repo_name': 'mCornish/blog', 'id': '531931936be565d6686ea08b70c338b735000891', 'size': '5556', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'docs/tag/Lesson/index.html', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '66560'}, {'name': 'HTML', 'bytes': '11134'}, {'name': 'JavaScript', 'bytes': '2008'}, {'name': 'Ruby', 'bytes': '1026'}]} |
package eu.ramich.popularmovies.ui;
import android.content.Intent;
import android.database.Cursor;
import android.graphics.Bitmap;
import android.graphics.drawable.BitmapDrawable;
import android.net.Uri;
import android.os.Bundle;
import android.support.annotation.Nullable;
import android.support.design.widget.AppBarLayout;
import android.support.design.widget.CollapsingToolbarLayout;
import android.support.design.widget.FloatingActionButton;
import android.support.design.widget.Snackbar;
import android.support.v4.app.Fragment;
import android.support.v4.app.LoaderManager;
import android.support.v4.content.CursorLoader;
import android.support.v4.content.Loader;
import android.support.v7.app.AppCompatActivity;
import android.support.v7.graphics.Palette;
import android.support.v7.widget.LinearLayoutManager;
import android.support.v7.widget.RecyclerView;
import android.support.v7.widget.Toolbar;
import android.text.TextUtils;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ImageView;
import android.widget.TextView;
import com.squareup.picasso.Callback;
import com.squareup.picasso.Picasso;
import java.util.Locale;
import butterknife.BindView;
import butterknife.ButterKnife;
import eu.ramich.popularmovies.R;
import eu.ramich.popularmovies.adapter.ReviewAdapter;
import eu.ramich.popularmovies.adapter.TrailerAdapter;
import eu.ramich.popularmovies.data.MovieContract;
import eu.ramich.popularmovies.sync.PopularMoviesIntentService;
import eu.ramich.popularmovies.sync.PopularMoviesSyncTasks;
import eu.ramich.popularmovies.utilities.NetworkUtils;
import eu.ramich.popularmovies.utilities.PopularMoviesUtils;
public class MovieDetailsFragment extends Fragment
implements LoaderManager.LoaderCallbacks<Cursor>,
TrailerAdapter.TrailerAdapterOnClickHandler,
ReviewAdapter.ReviewAdapterOnClickHandler {
private static final String TAG = MovieDetailsFragment.class.getSimpleName();
private TrailerAdapter mTrailerAdapter;
private ReviewAdapter mReviewAdapter;
@BindView(R.id.rv_trailer) RecyclerView mTrailerRecyclerView;
@BindView(R.id.rv_review) RecyclerView mReviewRecyclerView;
@BindView(R.id.iv_movie_backdrop) ImageView movieBackdrop;
@BindView(R.id.toolbar_layout) CollapsingToolbarLayout cToolbarLayout;
@BindView(R.id.app_bar) AppBarLayout appBarLayout;
@BindView(R.id.tv_movie_title_detail) TextView movieTitle;
@BindView(R.id.tv_movie_release_date) TextView movieRelease;
@BindView(R.id.tv_movie_overview) TextView movieOverview;
@BindView(R.id.iv_movie_thumbnail) ImageView movieThumbnail;
@BindView(R.id.tv_movie_average_rating) TextView movieAverageRating;
@BindView(R.id.fab_fav) FloatingActionButton fav;
public static final String[] DETAIL_MOVIE_PROJECTION = {
MovieContract.MovieEntry.COLUMN_MOVIE_ID,
MovieContract.MovieEntry.COLUMN_ORIGINAL_TITLE,
MovieContract.MovieEntry.COLUMN_TITLE,
MovieContract.MovieEntry.COLUMN_BACKDROP_PATH,
MovieContract.MovieEntry.COLUMN_POSTER_PATH,
MovieContract.MovieEntry.COLUMN_VOTE_AVERAGE,
MovieContract.MovieEntry.COLUMN_RELEASE_DATE,
MovieContract.MovieEntry.COLUMN_OVERVIEW,
MovieContract.MovieEntry.COLUMN_FAVORITE
};
public static final int INDEX_MOVIE_ID = 0;
public static final int INDEX_ORIGINAL_TITLE = 1;
public static final int INDEX_TITLE = 2;
public static final int INDEX_BACKDROP_PATH = 3;
public static final int INDEX_POSTER_PATH = 4;
public static final int INDEX_VOTE_AVERAGE = 5;
public static final int INDEX_RELEASE_DATE = 6;
public static final int INDEX_OVERVIEW = 7;
public static final int INDEX_FAVOURITE = 8;
public static final String[] DETAIL_TRAILER_PROJECTION = {
MovieContract.TrailerEntry.COLUMN_KEY,
MovieContract.TrailerEntry.COLUMN_NAME
};
public static final int INDEX_TRAILER_KEY = 0;
public static final int INDEX_TRAILER_NAME = 1;
public static final String[] DETAIL_REVIEW_PROJECTION = {
MovieContract.ReviewEntry.COLUMN_AUTHOR,
MovieContract.ReviewEntry.COLUMN_CONTENT
};
public static final int INDEX_REVIEW_AUTHOR = 0;
public static final int INDEX_REVIEW_CONTENT = 1;
private Uri mUri;
private String mTitle;
private static final int ID_MOVIE_LOADER = 7;
private static final int ID_TRAILER_LOADER = 8;
private static final int ID_REVIEWS_LOADER = 9;
public static MovieDetailsFragment newInstance(Uri uriForMovie) {
MovieDetailsFragment f = new MovieDetailsFragment();
Bundle args = new Bundle();
args.putParcelable("uri", uriForMovie);
f.setArguments(args);
return f;
}
public Uri getShownMovieUri() {
return getArguments().getParcelable("uri");
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.fragment_movie_detail, container, false);
ButterKnife.bind(this, view);
return view;
}
@Override
public void onActivityCreated(@Nullable Bundle savedInstanceState) {
super.onActivityCreated(savedInstanceState);
if (savedInstanceState != null) {
mUri = savedInstanceState.getParcelable("uri");
} else if (PopularMoviesUtils.isTablet()) {
mUri = getArguments().getParcelable("uri");
} else {
mUri = getActivity().getIntent().getData();
}
if (mUri == null)
throw new NullPointerException("URI for MovieDetailsActivity cannot be null");
appBarLayout.addOnOffsetChangedListener(new AppBarLayout.OnOffsetChangedListener() {
@Override
public void onOffsetChanged(AppBarLayout appBarLayout, int verticalOffset) {
if (appBarLayout.getTotalScrollRange() * -1 == verticalOffset) {
cToolbarLayout.setTitle(mTitle);
} else {
cToolbarLayout.setTitle(" ");
}
}
});
if (!PopularMoviesUtils.isTablet()) {
Toolbar toolbar = (Toolbar) getActivity().findViewById(R.id.toolbar);
((AppCompatActivity) getActivity()).setSupportActionBar(toolbar);
if (((AppCompatActivity) getActivity()).getSupportActionBar() != null)
((AppCompatActivity) getActivity()).getSupportActionBar().setDisplayHomeAsUpEnabled(true);
}
fav.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Intent favIntent = new Intent(getActivity(), PopularMoviesIntentService.class);
String message;
if (fav.getTag().equals(R.drawable.ic_favorite_border)) {
favIntent.setAction(PopularMoviesSyncTasks.ACTION_ADD_TO_FAV_MOVIES);
message = getString(R.string.favorite_add);
} else if (fav.getTag().equals(R.drawable.ic_favorite)) {
favIntent.setAction(PopularMoviesSyncTasks.ACTION_REMOVE_FROM_FAV_MOVIES);
message = getString(R.string.favorite_remove);
} else {
return;
}
favIntent.putExtra("id", mUri.getLastPathSegment());
getActivity().startService(favIntent);
Snackbar.make(view, message, Snackbar.LENGTH_LONG).show();
}
});
mTrailerAdapter = new TrailerAdapter(this);
mTrailerRecyclerView.setAdapter(mTrailerAdapter);
mTrailerRecyclerView.setLayoutManager(new LinearLayoutManager(getActivity(),
LinearLayoutManager.HORIZONTAL, false));
mTrailerRecyclerView.setHasFixedSize(true);
mReviewAdapter = new ReviewAdapter(this);
mReviewRecyclerView.setAdapter(mReviewAdapter);
mReviewRecyclerView.setLayoutManager(new LinearLayoutManager(getActivity(),
LinearLayoutManager.VERTICAL, false));
mReviewRecyclerView.setHasFixedSize(true);
Intent intent = new Intent(getActivity(), PopularMoviesIntentService.class);
intent.setAction(PopularMoviesSyncTasks.ACTION_SYNC_VIDEO_LIST);
intent.putExtra("id", mUri.getLastPathSegment());
getActivity().startService(intent);
Intent reviewIntent = new Intent(getActivity(), PopularMoviesIntentService.class);
reviewIntent.setAction(PopularMoviesSyncTasks.ACTION_SYNC_REVIEW_LIST);
reviewIntent.putExtra("id", mUri.getLastPathSegment());
getActivity().startService(reviewIntent);
getLoaderManager().initLoader(ID_MOVIE_LOADER, null, this);
getLoaderManager().initLoader(ID_TRAILER_LOADER, null, this);
getLoaderManager().initLoader(ID_REVIEWS_LOADER, null, this);
}
@Override
public Loader<Cursor> onCreateLoader(int id, Bundle args) {
String selection;
String[] selectionArgs;
switch (id) {
case ID_MOVIE_LOADER:
return new CursorLoader(getActivity(),
mUri,
DETAIL_MOVIE_PROJECTION,
null,
null,
null);
case ID_TRAILER_LOADER:
selection = MovieContract.TrailerEntry.trailerSelection();
selectionArgs = MovieContract.TrailerEntry
.trailerSelectionArgs(mUri.getLastPathSegment());
return new CursorLoader(getActivity(),
MovieContract.TrailerEntry.CONTENT_URI,
DETAIL_TRAILER_PROJECTION,
selection,
selectionArgs,
null);
case ID_REVIEWS_LOADER:
selection = MovieContract.ReviewEntry.COLUMN_MOVIE_ID + " = ?";
selectionArgs = new String[]{mUri.getLastPathSegment()};
return new CursorLoader(getActivity(),
MovieContract.ReviewEntry.CONTENT_URI,
DETAIL_REVIEW_PROJECTION,
selection,
selectionArgs,
null);
default:
throw new RuntimeException("Loader Not Implemented: " + id);
}
}
@Override
public void onLoadFinished(Loader<Cursor> loader, Cursor data) {
boolean cursorHasValidData = false;
if (data != null && data.moveToFirst()) {
cursorHasValidData = true;
}
if (!cursorHasValidData)
return;
switch (loader.getId()) {
case ID_MOVIE_LOADER:
mTitle = data.getString(INDEX_TITLE);
movieTitle.setText(data.getString(INDEX_TITLE));
movieOverview.setText(data.getString(INDEX_OVERVIEW));
movieRelease.setText(PopularMoviesUtils.normalizeDate(data.getString(INDEX_RELEASE_DATE)));
movieAverageRating.setText(String.format(Locale.getDefault(),
"%.1f/10", data.getDouble(INDEX_VOTE_AVERAGE)));
if (data.getInt(INDEX_FAVOURITE) == 1) {
fav.setImageResource(R.drawable.ic_favorite);
fav.setTag(R.drawable.ic_favorite);
} else {
fav.setImageResource(R.drawable.ic_favorite_border);
fav.setTag(R.drawable.ic_favorite_border);
}
Picasso.with(getActivity())
.load(NetworkUtils.buildPosterUrl(data.getString(INDEX_POSTER_PATH),
PopularMoviesUtils.getPosterWidth(true)).toString())
.into(movieThumbnail);
Picasso.with(getActivity())
.load(NetworkUtils.buildPosterUrl(data.getString(INDEX_BACKDROP_PATH),
PopularMoviesUtils.getPosterWidth(false)).toString())
.into(movieBackdrop, new Callback() {
@Override
public void onSuccess() {
Bitmap bitmap = ((BitmapDrawable) movieBackdrop.getDrawable())
.getBitmap();
Palette.from(bitmap).generate(new Palette.PaletteAsyncListener() {
@Override
public void onGenerated(Palette palette) {
Palette.Swatch swatch = palette.getDominantSwatch();
if (swatch == null) {
return;
}
int color = swatch.getRgb();
cToolbarLayout.setBackgroundColor(color);
cToolbarLayout.setStatusBarScrimColor(
palette.getDarkVibrantColor(color));
cToolbarLayout.setContentScrimColor(
palette.getVibrantColor(color));
}
});
}
@Override
public void onError() {
Log.e(TAG, "Error occured");
}
});
break;
case ID_TRAILER_LOADER:
mTrailerAdapter.swapCursor(data);
break;
case ID_REVIEWS_LOADER:
mReviewAdapter.swapCursor(data);
break;
}
}
@Override
public void onLoaderReset(Loader<Cursor> loader) {
switch (loader.getId()) {
case ID_TRAILER_LOADER:
mTrailerAdapter.swapCursor(null);
break;
case ID_REVIEWS_LOADER:
mReviewAdapter.swapCursor(null);
break;
}
}
@Override
public void onClick(String trailerKey) {
Uri trailerUri = NetworkUtils.buildTrailerUri(trailerKey);
Intent trailerIntent = new Intent(Intent.ACTION_VIEW, trailerUri);
if (trailerIntent.resolveActivity(getActivity().getPackageManager()) != null) {
startActivity(trailerIntent);
}
}
@Override
public void onClick(ReviewAdapter.ReviewAdapterViewHolder rA) {
if (!rA.isExpanded) {
rA.reviewContent.setMaxLines(Integer.MAX_VALUE);
rA.reviewContent.setEllipsize(null);
rA.reviewArrow.setVisibility(View.VISIBLE);
} else {
rA.reviewContent.setMaxLines(3);
rA.reviewContent.setEllipsize(TextUtils.TruncateAt.END);
rA.reviewArrow.setVisibility(View.GONE);
}
rA.isExpanded = !rA.isExpanded;
}
@Override
public void onSaveInstanceState(Bundle outState) {
super.onSaveInstanceState(outState);
outState.putParcelable("uri", mUri);
}
}
| {'content_hash': '2c43256dd374f1ca495d9cee4b59ccc1', 'timestamp': '', 'source': 'github', 'line_count': 386, 'max_line_length': 107, 'avg_line_length': 40.015544041450774, 'alnum_prop': 0.6131037161724718, 'repo_name': 'Shenkaa/Popular-Movies', 'id': '2f9d8003a4b4b246a53ae7d31d166a11471754fe', 'size': '15446', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'app/src/main/java/eu/ramich/popularmovies/ui/MovieDetailsFragment.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Java', 'bytes': '90997'}]} |
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using OpenTK.Graphics.OpenGL;
namespace Treton.Graphics
{
public class Mesh : IDisposable
{
public int Handle { get; private set; }
public Buffer VertexBuffer { get; private set; }
public Buffer IndexBuffer { get; private set; }
public SubMesh[] SubMeshes { get; private set; }
public Material[] Materials;
public Mesh(VertexFormat vertexFormat, byte[] vertexData, byte[] indexData, SubMesh[] subMeshes, Material[] materials, bool mutable = false)
{
if (vertexFormat == null)
throw new ArgumentNullException("vertexFormat");
if (vertexData == null)
throw new ArgumentNullException("vertexData");
if (indexData == null)
throw new ArgumentNullException("indexData");
if (subMeshes == null)
throw new ArgumentNullException("subMeshes");
if (materials == null)
throw new ArgumentNullException("materials");
if (subMeshes.Length != materials.Length)
throw new ArgumentException("subMeshes <-> materials length mismatch");
Handle = GL.GenVertexArray();
SubMeshes = subMeshes;
Materials = materials;
VertexBuffer = new Buffer(BufferTarget.ArrayBuffer, vertexFormat, mutable);
IndexBuffer = new Buffer(BufferTarget.ElementArrayBuffer, mutable);
VertexBuffer.SetData(vertexData);
IndexBuffer.SetData(indexData);
GL.BindVertexArray(Handle);
GL.BindBuffer(IndexBuffer.Target, IndexBuffer.Handle);
GL.BindVertexArray(0);
GL.Ext.VertexArrayBindVertexBuffer(Handle, 0, VertexBuffer.Handle, IntPtr.Zero, vertexFormat.Size);
for (var v = 0; v < vertexFormat.Elements.Length; v++)
{
var element = vertexFormat.Elements[v];
var index = (int)element.Semantic;
GL.Ext.EnableVertexArrayAttrib(Handle, index);
GL.Ext.VertexArrayVertexAttribBinding(Handle, index, 0);
GL.Ext.VertexArrayVertexAttribFormat(Handle, index, element.Count, (ExtDirectStateAccess)element.Type, false, element.Offset);
GL.Ext.VertexArrayVertexBindingDivisor(Handle, 0, element.Divisor);
}
}
public void Dispose()
{
Dispose(true);
GC.SuppressFinalize(this);
}
~Mesh()
{
Dispose(false);
}
protected virtual void Dispose(bool disposing)
{
if (Handle != 0)
{
GL.DeleteVertexArray(Handle);
Handle = 0;
}
if (VertexBuffer != null)
{
VertexBuffer.Dispose();
}
if (IndexBuffer != null)
{
IndexBuffer.Dispose();
}
}
public void Bind()
{
GL.BindVertexArray(Handle);
}
public struct SubMesh
{
public int Offset;
public int Count;
public SubMesh(int offset, int count)
{
Offset = offset;
Count = count;
}
}
}
}
| {'content_hash': '1f89c26351a22582720fd92233dfc8d7', 'timestamp': '', 'source': 'github', 'line_count': 109, 'max_line_length': 142, 'avg_line_length': 24.972477064220183, 'alnum_prop': 0.6987509184423218, 'repo_name': 'johang88/Treton', 'id': 'b808c0edbd97622edb59eb8939a58203595a86e1', 'size': '2724', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'Source/Treton/Graphics/Mesh.cs', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'C#', 'bytes': '86596'}, {'name': 'F#', 'bytes': '999'}, {'name': 'GLSL', 'bytes': '5748'}, {'name': 'Shell', 'bytes': '286'}, {'name': 'Verilog', 'bytes': '276'}]} |
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>class Rake::EarlyTime - rake-12.3.2 Documentation</title>
<script type="text/javascript">
var rdoc_rel_prefix = "../";
</script>
<script src="../js/jquery.js"></script>
<script src="../js/darkfish.js"></script>
<link href="../css/fonts.css" rel="stylesheet">
<link href="../css/rdoc.css" rel="stylesheet">
<body id="top" role="document" class="class">
<nav role="navigation">
<div id="project-navigation">
<div id="home-section" role="region" title="Quick navigation" class="nav-section">
<h2>
<a href="../index.html" rel="home">Home</a>
</h2>
<div id="table-of-contents-navigation">
<a href="../table_of_contents.html#pages">Pages</a>
<a href="../table_of_contents.html#classes">Classes</a>
<a href="../table_of_contents.html#methods">Methods</a>
</div>
</div>
<div id="search-section" role="search" class="project-section initially-hidden">
<form action="#" method="get" accept-charset="utf-8">
<div id="search-field-wrapper">
<input id="search-field" role="combobox" aria-label="Search"
aria-autocomplete="list" aria-controls="search-results"
type="text" name="search" placeholder="Search" spellcheck="false"
title="Type to search, Up and Down to navigate, Enter to load">
</div>
<ul id="search-results" aria-label="Search Results"
aria-busy="false" aria-expanded="false"
aria-atomic="false" class="initially-hidden"></ul>
</form>
</div>
</div>
<div id="class-metadata">
<div id="parent-class-section" class="nav-section">
<h3>Parent</h3>
<p class="link"><a href="../Object.html">Object</a>
</div>
<div id="includes-section" class="nav-section">
<h3>Included Modules</h3>
<ul class="link-list">
<li><span class="include">Comparable</span>
<li><span class="include">Singleton</span>
</ul>
</div>
<!-- Method Quickref -->
<div id="method-list-section" class="nav-section">
<h3>Methods</h3>
<ul class="link-list" role="directory">
<li ><a href="#method-i-3C-3D-3E">#<=></a>
</ul>
</div>
</div>
</nav>
<main role="main" aria-labelledby="class-Rake::EarlyTime">
<h1 id="class-Rake::EarlyTime" class="class">
class Rake::EarlyTime
</h1>
<section class="description">
<p><a href="EarlyTime.html">EarlyTime</a> is a fake timestamp that occurs
<em>before</em> any other time value.</p>
</section>
<section id="5Buntitled-5D" class="documentation-section">
<section id="public-instance-5Buntitled-5D-method-details" class="method-section">
<header>
<h3>Public Instance Methods</h3>
</header>
<div id="method-i-3C-3D-3E" class="method-detail ">
<div class="method-heading">
<span class="method-name"><=></span><span
class="method-args">(other)</span>
<span class="method-click-advice">click to toggle source</span>
</div>
<div class="method-description">
<p>The <a href="EarlyTime.html">EarlyTime</a> always comes before
<code>other</code>!</p>
<div class="method-source-code" id="3C-3D-3E-source">
<pre><span class="ruby-comment"># File lib/rake/early_time.rb, line 12</span>
<span class="ruby-keyword">def</span> <span class="ruby-operator"><=></span>(<span class="ruby-identifier">other</span>)
<span class="ruby-value">-1</span>
<span class="ruby-keyword">end</span></pre>
</div>
</div>
</div>
</section>
</section>
</main>
<footer id="validator-badges" role="contentinfo">
<p><a href="http://validator.w3.org/check/referer">Validate</a>
<p>Generated by <a href="http://docs.seattlerb.org/rdoc/">RDoc</a> 4.2.1.
<p>Based on <a href="http://deveiate.org/projects/Darkfish-RDoc/">Darkfish</a> by <a href="http://deveiate.org">Michael Granger</a>.
</footer>
| {'content_hash': '6db7bf464402d5af3144160f7fdc33ed', 'timestamp': '', 'source': 'github', 'line_count': 172, 'max_line_length': 134, 'avg_line_length': 23.91860465116279, 'alnum_prop': 0.5947982498784637, 'repo_name': 'Celyagd/celyagd.github.io', 'id': '6c7b94e1ccc5c6f4c665c0e91a728481ad75af28', 'size': '4114', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'stakes-jekyll/doc/rake-12.3.2/rdoc/Rake/EarlyTime.html', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'C', 'bytes': '1375233'}, {'name': 'C++', 'bytes': '71485'}, {'name': 'CMake', 'bytes': '314'}, {'name': 'CSS', 'bytes': '168680'}, {'name': 'HTML', 'bytes': '7735250'}, {'name': 'Java', 'bytes': '134091'}, {'name': 'JavaScript', 'bytes': '196815'}, {'name': 'PowerShell', 'bytes': '238'}, {'name': 'REXX', 'bytes': '1941'}, {'name': 'Ragel', 'bytes': '56865'}, {'name': 'Roff', 'bytes': '3725'}, {'name': 'Ruby', 'bytes': '2708464'}, {'name': 'Shell', 'bytes': '842'}, {'name': 'Yacc', 'bytes': '7664'}]} |
import {Command, flags} from '@heroku-cli/command'
import {cli} from 'cli-ux'
import {Dyno, Release} from '@heroku-cli/schema'
export default class Wait extends Command {
static description = 'wait for all dynos to be running latest version after a release'
static flags = {
app: flags.app({required: true}),
remote: flags.remote(),
type: flags.string({
char: 't',
description: 'wait for one specific dyno type',
}),
'wait-interval': flags.integer({
char: 'w',
description: 'how frequently to poll in seconds (to avoid hitting Heroku API rate limits)',
parse: input => {
const w = parseInt(input, 10)
if (w < 10) {
cli.error('wait-interval must be at least 10', {exit: 1})
}
return w
},
default: 10,
}),
'with-run': flags.boolean({
char: 'R',
description: 'whether to wait for one-off run dynos',
exclusive: ['type'],
}),
}
async run() {
const {flags} = this.parse(Wait)
const {body: releases} = await this.heroku.request<Release[]>(`/apps/${flags.app}/releases`, {
partial: true,
headers: {
Range: 'version ..; max=1, order=desc',
},
})
if (releases.length === 0) {
this.warn(`App ${flags.app} has no releases`)
return
}
const latestRelease = releases[0]
let released = true
const interval = flags['wait-interval'] as number
while (1 as any) {
// eslint-disable-next-line no-await-in-loop
const {body: dynos} = await this.heroku.get<Dyno[]>(`/apps/${flags.app}/dynos`)
const relevantDynos = dynos
.filter(dyno => dyno.type !== 'release')
.filter(dyno => flags['with-run'] || dyno.type !== 'run')
.filter(dyno => !flags.type || dyno.type === flags.type)
const onLatest = relevantDynos.filter((dyno: Dyno) => {
return dyno.state === 'up' &&
latestRelease.version !== undefined &&
dyno.release !== undefined &&
dyno.release.version !== undefined &&
dyno.release.version >= latestRelease.version
})
const releasedFraction = `${onLatest.length} / ${relevantDynos.length}`
if (onLatest.length === relevantDynos.length) {
if (!released) {
cli.action.stop(`${releasedFraction}, done`)
}
break
}
if (released) {
released = false
cli.action.start(`Waiting for every dyno to be running v${latestRelease.version}`)
}
cli.action.status = releasedFraction
// eslint-disable-next-line no-await-in-loop
await cli.wait(interval * 1000)
}
}
}
| {'content_hash': '99477e3e2ddfef014be44817a5512535', 'timestamp': '', 'source': 'github', 'line_count': 89, 'max_line_length': 98, 'avg_line_length': 29.831460674157302, 'alnum_prop': 0.584180790960452, 'repo_name': 'heroku/heroku-cli', 'id': '7d364fb3dd3e811dc38533fb288ebf5aa1e3d32d', 'size': '2655', 'binary': False, 'copies': '2', 'ref': 'refs/heads/master', 'path': 'packages/ps/src/commands/ps/wait.ts', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Batchfile', 'bytes': '239'}, {'name': 'Go', 'bytes': '87715'}, {'name': 'JavaScript', 'bytes': '4155'}, {'name': 'Ruby', 'bytes': '4575'}, {'name': 'Shell', 'bytes': '434'}]} |
/**
* Creates a rule that will be applied when an MDC-Web component is within the context of an RTL layout.
*
* Usage Example:
* ```scss
* .mdc-foo {
* position: absolute;
* left: 0;
*
* @include mdc-rtl {
* left: auto;
* right: 0;
* }
*
* &__bar {
* margin-left: 4px;
* @include mdc-rtl(".mdc-foo") {
* margin-left: auto;
* margin-right: 4px;
* }
* }
* }
*
* .mdc-foo--mod {
* padding-left: 4px;
*
* @include mdc-rtl {
* padding-left: auto;
* padding-right: 4px;
* }
* }
* ```
*
* Note that this works by checking for [dir="rtl"] on an ancestor element. While this will work
* in most cases, it will in some cases lead to false negatives, e.g.
*
* ```html
* <html dir="rtl">
* <!-- ... -->
* <div dir="ltr">
* <div class="mdc-foo">Styled incorrectly as RTL!</div>
* </div>
* </html>
* ```
*
* In the future, selectors such as :dir (http://mdn.io/:dir) will help us mitigate this.
*/
/**
* Takes a base box-model property - e.g. margin / border / padding - along with a default
* direction and value, and emits rules which apply the value to the
* "<base-property>-<default-direction>" property by default, but flips the direction
* when within an RTL context.
*
* For example:
*
* ```scss
* .mdc-foo {
* @include mdc-rtl-reflexive-box(margin, left, 8px);
* }
* ```
* is equivalent to:
*
* ```scss
* .mdc-foo {
* margin-left: 8px;
*
* @include mdc-rtl {
* margin-right: 8px;
* margin-left: 0;
* }
* }
* ```
* whereas:
*
* ```scss
* .mdc-foo {
* @include mdc-rtl-reflexive-box(margin, right, 8px);
* }
* ```
* is equivalent to:
*
* ```scss
* .mdc-foo {
* margin-right: 8px;
*
* @include mdc-rtl {
* margin-right: 0;
* margin-left: 8px;
* }
* }
* ```
*
* You can also pass a 4th optional $root-selector argument which will be forwarded to `mdc-rtl`,
* e.g. `@include mdc-rtl-reflexive-box-property(margin, left, 8px, ".mdc-component")`.
*
* Note that this function will always zero out the original value in an RTL context. If you're
* trying to flip the values, use mdc-rtl-reflexive-property().
*/
/**
* Takes a base property and emits rules that assign <base-property>-left to <left-value> and
* <base-property>-right to <right-value> in a LTR context, and vice versa in a RTL context.
* For example:
*
* ```scss
* .mdc-foo {
* @include mdc-rtl-reflexive-property(margin, auto, 12px);
* }
* ```
* is equivalent to:
*
* ```scss
* .mdc-foo {
* margin-left: auto;
* margin-right: 12px;
*
* @include mdc-rtl {
* margin-left: 12px;
* margin-right: auto;
* }
* }
* ```
*
* A 4th optional $root-selector argument can be given, which will be passed to `mdc-rtl`.
*/
/**
* Takes an argument specifying a horizontal position property (either "left" or "right") as well
* as a value, and applies that value to the specified position in a LTR context, and flips it in a
* RTL context. For example:
*
* ```scss
* .mdc-foo {
* @include mdc-rtl-reflexive-position(left, 0);
* position: absolute;
* }
* ```
* is equivalent to:
*
* ```scss
* .mdc-foo {
* position: absolute;
* left: 0;
* right: initial;
*
* @include mdc-rtl {
* right: 0;
* left: initial;
* }
* }
* ```
* An optional third $root-selector argument may also be given, which is passed to `mdc-rtl`.
*/
/*
Precomputed linear color channel values, for use in contrast calculations.
See https://www.w3.org/TR/WCAG20-TECHS/G17.html#G17-tests
Algorithm, for c in 0 to 255:
f(c) {
c = c / 255;
return c < 0.03928 ? c / 12.92 : Math.pow((c + 0.055) / 1.055, 2.4);
}
This lookup table is needed since there is no `pow` in SASS.
*/
/**
* Calculate the luminance for a color.
* See https://www.w3.org/TR/WCAG20-TECHS/G17.html#G17-tests
*/
/**
* Calculate the contrast ratio between two colors.
* See https://www.w3.org/TR/WCAG20-TECHS/G17.html#G17-tests
*/
/**
* Determine whether to use dark or light text on top of given color.
* Returns "dark" for dark text and "light" for light text.
*/
/*
Main theme colors.
If you're a user customizing your color scheme in SASS, these are probably the only variables you need to change.
*/
/* Indigo 500 */
/* Pink A200 */
/* White */
/* Which set of text colors to use for each main theme color (light or dark) */
/* Text colors according to light vs dark and text type */
/* Primary text colors for each of the theme colors */
/*
Precomputed linear color channel values, for use in contrast calculations.
See https://www.w3.org/TR/WCAG20-TECHS/G17.html#G17-tests
Algorithm, for c in 0 to 255:
f(c) {
c = c / 255;
return c < 0.03928 ? c / 12.92 : Math.pow((c + 0.055) / 1.055, 2.4);
}
This lookup table is needed since there is no `pow` in SASS.
*/
/**
* Calculate the luminance for a color.
* See https://www.w3.org/TR/WCAG20-TECHS/G17.html#G17-tests
*/
/**
* Calculate the contrast ratio between two colors.
* See https://www.w3.org/TR/WCAG20-TECHS/G17.html#G17-tests
*/
/**
* Determine whether to use dark or light text on top of given color.
* Returns "dark" for dark text and "light" for light text.
*/
/*
Main theme colors.
If you're a user customizing your color scheme in SASS, these are probably the only variables you need to change.
*/
/* Indigo 500 */
/* Pink A200 */
/* White */
/* Which set of text colors to use for each main theme color (light or dark) */
/* Text colors according to light vs dark and text type */
/* Primary text colors for each of the theme colors */
/**
* Applies the correct theme color style to the specified property.
* $property is typically color or background-color, but can be any CSS property that accepts color values.
* $style should be one of the map keys in $mdc-theme-property-values (_variables.scss).
*/
/**
* Creates a rule to be used in MDC-Web components for dark theming, and applies the provided contents.
* Should provide the $root-selector option if applied to anything other than the root selector.
* When used with a modifier class, provide a second argument of `true` for the $compound parameter
* to specify that this should be attached as a compound class.
*
* Usage example:
*
* ```scss
* .mdc-foo {
* color: black;
*
* @include mdc-theme-dark {
* color: white;
* }
*
* &__bar {
* background: black;
*
* @include mdc-theme-dark(".mdc-foo") {
* background: white;
* }
* }
* }
*
* .mdc-foo--disabled {
* opacity: .38;
*
* @include mdc-theme-dark(".mdc-foo", true) {
* opacity: .5;
* }
* }
* ```
*/
/* TODO(sgomes): Figure out what to do about desktop font sizes. */
/* TODO(sgomes): Figure out what to do about i18n and i18n font sizes. */
/* TODO(sgomes): Figure out what to do about desktop font sizes. */
/* TODO(sgomes): Figure out what to do about i18n and i18n font sizes. */
.mdc-textfield {
font-family: Roboto, sans-serif;
-moz-osx-font-smoothing: grayscale;
-webkit-font-smoothing: antialiased;
font-size: 1rem;
letter-spacing: 0.04em;
display: inline-block;
margin-bottom: 8px;
will-change: opacity, transform, color; }
.mdc-textfield__input {
color: rgba(0, 0, 0, 0.87);
color: var(--mdc-theme-text-primary-on-light, rgba(0, 0, 0, 0.87));
padding: 0 0 8px;
border: none;
background: none;
font-size: inherit;
appearance: none; }
.mdc-textfield__input::placeholder {
color: rgba(0, 0, 0, 0.38);
color: var(--mdc-theme-text-hint-on-light, rgba(0, 0, 0, 0.38));
transition: color 180ms cubic-bezier(0.4, 0, 0.2, 1);
opacity: 1; }
.mdc-textfield__input:focus {
outline: none; }
.mdc-textfield__input:focus::placeholder {
color: rgba(0, 0, 0, 0.54);
color: var(--mdc-theme-text-secondary-on-light, rgba(0, 0, 0, 0.54)); }
.mdc-textfield__input:invalid {
box-shadow: none; }
.mdc-textfield__input--theme-dark,
.mdc-theme--dark .mdc-textfield__input {
color: white; }
.mdc-textfield__input--theme-dark::placeholder,
.mdc-theme--dark .mdc-textfield__input::placeholder {
color: rgba(255, 255, 255, 0.5);
color: var(--mdc-theme-text-hint-on-dark, rgba(255, 255, 255, 0.5)); }
.mdc-textfield__input--theme-dark:focus::placeholder,
.mdc-theme--dark .mdc-textfield__input:focus::placeholder {
color: rgba(255, 255, 255, 0.7);
color: var(--mdc-theme-text-secondary-on-dark, rgba(255, 255, 255, 0.7)); }
.mdc-textfield__label {
color: rgba(0, 0, 0, 0.38);
color: var(--mdc-theme-text-hint-on-light, rgba(0, 0, 0, 0.38));
position: absolute;
bottom: 8px;
left: 0;
transform-origin: left top;
transition: transform 180ms cubic-bezier(0.4, 0, 0.2, 1), color 180ms cubic-bezier(0.4, 0, 0.2, 1);
cursor: text; }
[dir="rtl"] .mdc-textfield .mdc-textfield__label,
.mdc-textfield[dir="rtl"] .mdc-textfield__label {
right: 0;
left: auto;
transform-origin: right top; }
.mdc-textfield--theme-dark .mdc-textfield__label,
.mdc-theme--dark .mdc-textfield__label {
color: rgba(255, 255, 255, 0.5);
color: var(--mdc-theme-text-hint-on-dark, rgba(255, 255, 255, 0.5)); }
.mdc-textfield__label--float-above {
transform: translateY(-100%) scale(0.75, 0.75);
cursor: auto; }
.mdc-textfield__input:-webkit-autofill + .mdc-textfield__label {
transform: translateY(-100%) scale(0.75, 0.75);
cursor: auto; }
.mdc-textfield--upgraded:not(.mdc-textfield--fullwidth) {
display: inline-flex;
position: relative;
box-sizing: border-box;
align-items: flex-end;
margin-top: 16px; }
.mdc-textfield--upgraded:not(.mdc-textfield--fullwidth):not(.mdc-textfield--multiline) {
height: 48px; }
.mdc-textfield--upgraded:not(.mdc-textfield--fullwidth):not(.mdc-textfield--multiline)::after {
position: absolute;
bottom: 0;
left: 0;
width: 100%;
height: 1px;
transform: translateY(50%) scaleY(1);
transform-origin: center bottom;
transition: background-color 180ms cubic-bezier(0.4, 0, 0.2, 1), transform 180ms cubic-bezier(0.4, 0, 0.2, 1);
background-color: rgba(0, 0, 0, 0.12);
content: ""; }
.mdc-textfield--theme-dark .mdc-textfield--upgraded:not(.mdc-textfield--fullwidth):not(.mdc-textfield--multiline)::after,
.mdc-theme--dark .mdc-textfield--upgraded:not(.mdc-textfield--fullwidth):not(.mdc-textfield--multiline)::after {
background-color: rgba(255, 255, 255, 0.12); }
.mdc-textfield--upgraded:not(.mdc-textfield--fullwidth) .mdc-textfield__label {
pointer-events: none; }
.mdc-textfield--focused.mdc-textfield--upgraded:not(.mdc-textfield--fullwidth):not(.mdc-textfield--multiline)::after {
background-color: #3f51b5;
background-color: var(--mdc-theme-primary, #3f51b5);
transform: translateY(100%) scaleY(2);
transition: transform 180ms cubic-bezier(0.4, 0, 0.2, 1); }
.mdc-textfield--theme-dark.mdc-textfield--focused.mdc-textfield--upgraded:not(.mdc-textfield--fullwidth):not(.mdc-textfield--multiline)::after,
.mdc-theme--dark .mdc-textfield--focused.mdc-textfield--upgraded:not(.mdc-textfield--fullwidth):not(.mdc-textfield--multiline)::after {
background-color: #3f51b5;
background-color: var(--mdc-theme-primary, #3f51b5);
transform: translateY(100%) scaleY(2);
transition: transform 180ms cubic-bezier(0.4, 0, 0.2, 1); }
.mdc-textfield--focused .mdc-textfield__label {
color: #3f51b5;
color: var(--mdc-theme-primary, #3f51b5); }
.mdc-textfield--theme-dark .mdc-textfield--focused .mdc-textfield__label,
.mdc-theme--dark .mdc-textfield--focused .mdc-textfield__label {
color: #3f51b5;
color: var(--mdc-theme-primary, #3f51b5); }
.mdc-textfield--dense {
margin-top: 12px;
margin-bottom: 4px;
font-size: .813rem; }
.mdc-textfield--dense .mdc-textfield__label--float-above {
transform: translateY(calc(-100% - 2px)) scale(0.923, 0.923); }
.mdc-textfield--invalid:not(.mdc-textfield--focused)::after, .mdc-textfield--invalid:not(.mdc-textfield--focused).mdc-textfield--upgraded::after {
background-color: #d50000; }
.mdc-textfield--invalid:not(.mdc-textfield--focused) .mdc-textfield__label {
color: #d50000; }
.mdc-textfield--theme-dark.mdc-textfield--invalid:not(.mdc-textfield--focused)::after, .mdc-textfield--theme-dark.mdc-textfield--invalid:not(.mdc-textfield--focused).mdc-textfield--upgraded::after,
.mdc-theme--dark .mdc-textfield--invalid:not(.mdc-textfield--focused)::after,
.mdc-theme--dark .mdc-textfield--invalid:not(.mdc-textfield--focused).mdc-textfield--upgraded::after {
background-color: #ff6e6e; }
.mdc-textfield--theme-dark.mdc-textfield--invalid:not(.mdc-textfield--focused) .mdc-textfield__label,
.mdc-theme--dark .mdc-textfield--invalid:not(.mdc-textfield--focused) .mdc-textfield__label {
color: #ff6e6e; }
.mdc-textfield--disabled {
border-bottom: 1px dotted rgba(35, 31, 32, 0.26); }
.mdc-textfield--disabled::after {
display: none; }
.mdc-textfield--disabled .mdc-textfield__input {
padding-bottom: 7px; }
.mdc-textfield--theme-dark.mdc-textfield--disabled,
.mdc-theme--dark .mdc-textfield--disabled {
border-bottom: 1px dotted rgba(255, 255, 255, 0.3); }
.mdc-textfield--disabled .mdc-textfield__input,
.mdc-textfield--disabled .mdc-textfield__label,
.mdc-textfield--disabled + .mdc-textfield-helptext {
color: rgba(0, 0, 0, 0.38);
color: var(--mdc-theme-text-disabled-on-light, rgba(0, 0, 0, 0.38)); }
.mdc-textfield--theme-dark .mdc-textfield--disabled .mdc-textfield__input,
.mdc-theme--dark .mdc-textfield--disabled .mdc-textfield__input, .mdc-textfield--theme-dark
.mdc-textfield--disabled .mdc-textfield__label,
.mdc-theme--dark
.mdc-textfield--disabled .mdc-textfield__label {
color: rgba(255, 255, 255, 0.5);
color: var(--mdc-theme-text-disabled-on-dark, rgba(255, 255, 255, 0.5)); }
.mdc-textfield--theme-dark.mdc-textfield--disabled + .mdc-textfield-helptext,
.mdc-theme--dark .mdc-textfield--disabled + .mdc-textfield-helptext {
color: rgba(255, 255, 255, 0.5);
color: var(--mdc-theme-text-disabled-on-dark, rgba(255, 255, 255, 0.5)); }
.mdc-textfield--disabled .mdc-textfield__label {
bottom: 7px;
cursor: default; }
.mdc-textfield__input:required + .mdc-textfield__label::after {
margin-left: 1px;
content: "*"; }
.mdc-textfield--focused .mdc-textfield__input:required + .mdc-textfield__label::after {
color: #d50000; }
.mdc-textfield--focused .mdc-textfield--theme-dark .mdc-textfield__input:required + .mdc-textfield__label::after, .mdc-textfield--focused
.mdc-theme--dark .mdc-textfield__input:required + .mdc-textfield__label::after {
color: #ff6e6e; }
.mdc-textfield--multiline {
display: flex;
height: initial;
transition: none; }
.mdc-textfield--multiline::after {
content: initial; }
.mdc-textfield--multiline .mdc-textfield__input {
padding: 4px;
transition: border-color 180ms cubic-bezier(0.4, 0, 0.2, 1);
border: 1px solid rgba(0, 0, 0, 0.12);
border-radius: 2px; }
.mdc-textfield--theme-dark .mdc-textfield--multiline .mdc-textfield__input,
.mdc-theme--dark .mdc-textfield--multiline .mdc-textfield__input {
border-color: rgba(255, 255, 255, 0.12); }
.mdc-textfield--multiline .mdc-textfield__input:focus {
border-color: #3f51b5;
border-color: var(--mdc-theme-primary, #3f51b5); }
.mdc-textfield--multiline .mdc-textfield__input:invalid:not(:focus) {
border-color: #d50000; }
.mdc-textfield--theme-dark .mdc-textfield--multiline .mdc-textfield__input:invalid:not(:focus),
.mdc-theme--dark .mdc-textfield--multiline .mdc-textfield__input:invalid:not(:focus) {
border-color: #ff6e6e; }
.mdc-textfield--multiline .mdc-textfield__label {
top: 6px;
bottom: initial;
left: 4px; }
[dir="rtl"] .mdc-textfield--multiline .mdc-textfield--multiline .mdc-textfield__label,
.mdc-textfield--multiline[dir="rtl"] .mdc-textfield--multiline .mdc-textfield__label {
right: 4px;
left: auto; }
.mdc-textfield--multiline .mdc-textfield__label--float-above {
transform: translateY(calc(-100% - 6px)) scale(0.923, 0.923); }
.mdc-textfield--multiline.mdc-textfield--disabled {
border-bottom: none; }
.mdc-textfield--multiline.mdc-textfield--disabled .mdc-textfield__input {
border: 1px dotted rgba(35, 31, 32, 0.26); }
.mdc-textfield--theme-dark .mdc-textfield--multiline.mdc-textfield--disabled .mdc-textfield__input,
.mdc-theme--dark .mdc-textfield--multiline.mdc-textfield--disabled .mdc-textfield__input {
border-color: rgba(255, 255, 255, 0.3); }
.mdc-textfield--fullwidth {
display: block;
width: 100%;
box-sizing: border-box;
margin: 0;
border: none;
border-bottom: 1px solid rgba(0, 0, 0, 0.12);
outline: none; }
.mdc-textfield--fullwidth:not(.mdc-textfield--multiline) {
height: 56px; }
.mdc-textfield--fullwidth.mdc-textfield--multiline {
padding: 20px 0 0; }
.mdc-textfield--fullwidth.mdc-textfield--dense:not(.mdc-textfield--multiline) {
height: 48px; }
.mdc-textfield--fullwidth.mdc-textfield--dense.mdc-textfield--multiline {
padding: 16px 0 0; }
.mdc-textfield--fullwidth.mdc-textfield--disabled, .mdc-textfield--fullwidth.mdc-textfield--disabled.mdc-textfield--multiline {
border-bottom: 1px dotted rgba(0, 0, 0, 0.12); }
.mdc-textfield--fullwidth--theme-dark,
.mdc-theme--dark .mdc-textfield--fullwidth {
border-bottom: 1px solid rgba(255, 255, 255, 0.12); }
.mdc-textfield--fullwidth--theme-dark.mdc-textfield--disabled, .mdc-textfield--fullwidth--theme-dark.mdc-textfield--disabled.mdc-textfield--multiline,
.mdc-theme--dark .mdc-textfield--fullwidth.mdc-textfield--disabled,
.mdc-theme--dark .mdc-textfield--fullwidth.mdc-textfield--disabled.mdc-textfield--multiline {
border-bottom: 1px dotted rgba(255, 255, 255, 0.12); }
.mdc-textfield--fullwidth .mdc-textfield__input {
width: 100%;
height: 100%;
padding: 0;
resize: none;
border: none !important; }
.mdc-textfield:not(.mdc-textfield--upgraded):not(.mdc-textfield--multiline) .mdc-textfield__input {
transition: border-bottom-color 180ms cubic-bezier(0.4, 0, 0.2, 1);
border-bottom: 1px solid rgba(0, 0, 0, 0.12); }
.mdc-textfield:not(.mdc-textfield--upgraded) .mdc-textfield__input:focus {
border-color: #3f51b5;
border-color: var(--mdc-theme-primary, #3f51b5); }
.mdc-textfield:not(.mdc-textfield--upgraded) .mdc-textfield__input:disabled {
color: rgba(0, 0, 0, 0.38);
color: var(--mdc-theme-text-disabled-on-light, rgba(0, 0, 0, 0.38));
border-style: dotted;
border-color: rgba(35, 31, 32, 0.26); }
.mdc-textfield:not(.mdc-textfield--upgraded) .mdc-textfield__input:invalid:not(:focus) {
border-color: #d50000; }
.mdc-textfield--theme-dark:not(.mdc-textfield--upgraded) .mdc-textfield__input:not(:focus),
.mdc-theme--dark .mdc-textfield:not(.mdc-textfield--upgraded) .mdc-textfield__input:not(:focus) {
border-color: rgba(255, 255, 255, 0.12); }
.mdc-textfield--theme-dark:not(.mdc-textfield--upgraded) .mdc-textfield__input:disabled,
.mdc-theme--dark .mdc-textfield:not(.mdc-textfield--upgraded) .mdc-textfield__input:disabled {
color: rgba(255, 255, 255, 0.5);
color: var(--mdc-theme-text-disabled-on-dark, rgba(255, 255, 255, 0.5));
border-color: rgba(255, 255, 255, 0.3); }
.mdc-textfield--theme-dark:not(.mdc-textfield--upgraded) .mdc-textfield__input:invalid:not(:focus),
.mdc-theme--dark .mdc-textfield:not(.mdc-textfield--upgraded) .mdc-textfield__input:invalid:not(:focus) {
border-color: #ff6e6e; }
.mdc-textfield-helptext {
color: rgba(0, 0, 0, 0.38);
color: var(--mdc-theme-text-hint-on-light, rgba(0, 0, 0, 0.38));
margin: 0;
transition: opacity 180ms cubic-bezier(0.4, 0, 0.2, 1);
font-size: .75rem;
opacity: 0;
will-change: opacity; }
.mdc-textfield-helptext--theme-dark,
.mdc-theme--dark .mdc-textfield-helptext {
color: rgba(255, 255, 255, 0.5);
color: var(--mdc-theme-text-hint-on-dark, rgba(255, 255, 255, 0.5)); }
.mdc-textfield + .mdc-textfield-helptext {
margin-bottom: 8px; }
.mdc-textfield--dense + .mdc-textfield-helptext {
margin-bottom: 4px; }
.mdc-textfield--focused + .mdc-textfield-helptext:not(.mdc-textfield-helptext--validation-msg) {
opacity: 1; }
.mdc-textfield-helptext--persistent {
transition: none;
opacity: 1;
will-change: initial; }
.mdc-textfield--invalid + .mdc-textfield-helptext--validation-msg {
color: #d50000;
opacity: 1; }
.mdc-textfield--theme-dark.mdc-textfield--invalid + .mdc-textfield-helptext--validation-msg,
.mdc-theme--dark .mdc-textfield--invalid + .mdc-textfield-helptext--validation-msg {
color: #ff6e6e; }
.mdc-form-field > .mdc-textfield + label {
align-self: flex-start; }
| {'content_hash': 'c6c7df31c345e2f7d21c88c53424bacf', 'timestamp': '', 'source': 'github', 'line_count': 571, 'max_line_length': 197, 'avg_line_length': 36.63922942206655, 'alnum_prop': 0.6581903350700253, 'repo_name': 'BCM-Company/octohydra', 'id': 'ed5e311331e3b72fe7658989d1f2e7e573809a33', 'size': '21013', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'node_modules/@material/textfield/dist/mdc.textfield.css', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Batchfile', 'bytes': '547'}, {'name': 'CSS', 'bytes': '42690'}, {'name': 'HTML', 'bytes': '36989'}, {'name': 'JavaScript', 'bytes': '11271'}]} |
#include "tbb/blocked_range3d.h"
#include "harness_assert.h"
// First test as much as we can without including other headers.
// Doing so should catch problems arising from failing to include headers.
template<typename Tag>
class AbstractValueType {
AbstractValueType() {}
int value;
public:
template<typename OtherTag>
friend AbstractValueType<OtherTag> MakeAbstractValueType( int i );
template<typename OtherTag>
friend int GetValueOf( const AbstractValueType<OtherTag>& v ) ;
};
template<typename Tag>
AbstractValueType<Tag> MakeAbstractValueType( int i ) {
AbstractValueType<Tag> x;
x.value = i;
return x;
}
template<typename Tag>
int GetValueOf( const AbstractValueType<Tag>& v ) {return v.value;}
template<typename Tag>
bool operator<( const AbstractValueType<Tag>& u, const AbstractValueType<Tag>& v ) {
return GetValueOf(u)<GetValueOf(v);
}
template<typename Tag>
std::size_t operator-( const AbstractValueType<Tag>& u, const AbstractValueType<Tag>& v ) {
return GetValueOf(u)-GetValueOf(v);
}
template<typename Tag>
AbstractValueType<Tag> operator+( const AbstractValueType<Tag>& u, std::size_t offset ) {
return MakeAbstractValueType<Tag>(GetValueOf(u)+int(offset));
}
struct PageTag {};
struct RowTag {};
struct ColTag {};
static void SerialTest() {
typedef AbstractValueType<PageTag> page_type;
typedef AbstractValueType<RowTag> row_type;
typedef AbstractValueType<ColTag> col_type;
typedef tbb::blocked_range3d<page_type,row_type,col_type> range_type;
for( int page_x=-4; page_x<4; ++page_x ) {
for( int page_y=page_x; page_y<4; ++page_y ) {
page_type page_i = MakeAbstractValueType<PageTag>(page_x);
page_type page_j = MakeAbstractValueType<PageTag>(page_y);
for( int page_grain=1; page_grain<4; ++page_grain ) {
for( int row_x=-4; row_x<4; ++row_x ) {
for( int row_y=row_x; row_y<4; ++row_y ) {
row_type row_i = MakeAbstractValueType<RowTag>(row_x);
row_type row_j = MakeAbstractValueType<RowTag>(row_y);
for( int row_grain=1; row_grain<4; ++row_grain ) {
for( int col_x=-4; col_x<4; ++col_x ) {
for( int col_y=col_x; col_y<4; ++col_y ) {
col_type col_i = MakeAbstractValueType<ColTag>(col_x);
col_type col_j = MakeAbstractValueType<ColTag>(col_y);
for( int col_grain=1; col_grain<4; ++col_grain ) {
range_type r( page_i, page_j, page_grain, row_i, row_j, row_grain, col_i, col_j, col_grain );
AssertSameType( r.is_divisible(), true );
AssertSameType( r.empty(), true );
AssertSameType( static_cast<range_type::page_range_type::const_iterator*>(0), static_cast<page_type*>(0) );
AssertSameType( static_cast<range_type::row_range_type::const_iterator*>(0), static_cast<row_type*>(0) );
AssertSameType( static_cast<range_type::col_range_type::const_iterator*>(0), static_cast<col_type*>(0) );
AssertSameType( r.pages(), tbb::blocked_range<page_type>( page_i, page_j, 1 ));
AssertSameType( r.rows(), tbb::blocked_range<row_type>( row_i, row_j, 1 ));
AssertSameType( r.cols(), tbb::blocked_range<col_type>( col_i, col_j, 1 ));
ASSERT( r.empty()==(page_x==page_y||row_x==row_y||col_x==col_y), NULL );
ASSERT( r.is_divisible()==(page_y-page_x>page_grain||row_y-row_x>row_grain||col_y-col_x>col_grain), NULL );
if( r.is_divisible() ) {
range_type r2(r,tbb::split());
if( (GetValueOf(r2.pages().begin())==GetValueOf(r.pages().begin())) && (GetValueOf(r2.rows().begin())==GetValueOf(r.rows().begin())) ) {
ASSERT( GetValueOf(r2.pages().end())==GetValueOf(r.pages().end()), NULL );
ASSERT( GetValueOf(r2.rows().end())==GetValueOf(r.rows().end()), NULL );
ASSERT( GetValueOf(r2.cols().begin())==GetValueOf(r.cols().end()), NULL );
} else {
if ( (GetValueOf(r2.pages().begin())==GetValueOf(r.pages().begin())) && (GetValueOf(r2.cols().begin())==GetValueOf(r.cols().begin())) ) {
ASSERT( GetValueOf(r2.pages().end())==GetValueOf(r.pages().end()), NULL );
ASSERT( GetValueOf(r2.cols().end())==GetValueOf(r.cols().end()), NULL );
ASSERT( GetValueOf(r2.rows().begin())==GetValueOf(r.rows().end()), NULL );
} else {
ASSERT( GetValueOf(r2.rows().end())==GetValueOf(r.rows().end()), NULL );
ASSERT( GetValueOf(r2.cols().end())==GetValueOf(r.cols().end()), NULL );
ASSERT( GetValueOf(r2.pages().begin())==GetValueOf(r.pages().end()), NULL );
}
}
}
}
}
}
}
}
}
}
}
}
}
#include "tbb/parallel_for.h"
#include "harness.h"
const int N = 1<<5;
unsigned char Array[N][N][N];
struct Striker {
// Note: we use <int> here instead of <long> in order to test for problems similar to Quad 407676
void operator()( const tbb::blocked_range3d<int>& r ) const {
for( tbb::blocked_range<int>::const_iterator i=r.pages().begin(); i!=r.pages().end(); ++i )
for( tbb::blocked_range<int>::const_iterator j=r.rows().begin(); j!=r.rows().end(); ++j )
for( tbb::blocked_range<int>::const_iterator k=r.cols().begin(); k!=r.cols().end(); ++k )
++Array[i][j][k];
}
};
void ParallelTest() {
for( int i=0; i<N; i=i<3 ? i+1 : i*3 ) {
for( int j=0; j<N; j=j<3 ? j+1 : j*3 ) {
for( int k=0; k<N; k=k<3 ? k+1 : k*3 ) {
const tbb::blocked_range3d<int> r( 0, i, 5, 0, j, 3, 0, k, 1 );
tbb::parallel_for( r, Striker() );
for( int l=0; l<N; ++l ) {
for( int m=0; m<N; ++m ) {
for( int n=0; n<N; ++n ) {
ASSERT( Array[l][m][n]==(l<i && m<j && n<k), NULL );
Array[l][m][n] = 0;
}
}
}
}
}
}
}
#include "tbb/task_scheduler_init.h"
int TestMain () {
SerialTest();
for( int p=MinThread; p<=MaxThread; ++p ) {
tbb::task_scheduler_init init(p);
ParallelTest();
}
return Harness::Done;
}
| {'content_hash': 'd3856c0d319be83a0f77abfd175bd424', 'timestamp': '', 'source': 'github', 'line_count': 161, 'max_line_length': 185, 'avg_line_length': 47.08074534161491, 'alnum_prop': 0.4737467018469657, 'repo_name': 'Teaonly/easyLearning.js', 'id': '242fcfd97eeabc6589def56b434efb1974fc34df', 'size': '8196', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'TensorExpress/aten/src/ATen/cpu/tbb/tbb_remote/src/test/test_blocked_range3d.cpp', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'C', 'bytes': '1'}, {'name': 'C++', 'bytes': '11485'}, {'name': 'JavaScript', 'bytes': '100936'}, {'name': 'Jupyter Notebook', 'bytes': '213476'}, {'name': 'Lua', 'bytes': '17603'}, {'name': 'Python', 'bytes': '320'}, {'name': 'Shell', 'bytes': '375'}]} |
/* global describe, it */
/* eslint no-invalid-this: 0 */
process.env.NODE_ENV = "test";
const assertTransform = require("assert-transform");
const path = require("path");
const BABEL_OPTIONS = {
presets: [],
plugins: [
"syntax-jsx",
[path.join(__dirname, "..", "lib", "index.js"), {
"cssmodule": "path/to/classnames.css"
}]
]
};
const test = (type, babelOptions) => (testCase) => () =>
assertTransform(
path.join(__dirname, "fixtures", type, testCase, "actual.js"),
path.join(__dirname, "fixtures", type, testCase, "expected.js"),
babelOptions || BABEL_OPTIONS
);
describe("babel-plugin-react-cssmoduleify", () => {
["jsx", "createElement", "compiled"].forEach((type) => {
describe(type, () => {
it("should transform simple literals", test(type)("string"));
it("should transform multiple-class string literals", test(type)("string-multiple"));
it("should transform JSXExpressionContainer values", test(type)("string-jsx-expression"));
it("should transform *.join(\" \") expressions", test(type)("array-join"));
it("should transform simple identifier expressions", test(type)("identifier"));
it("should transform a simple call expression", test(type)("call-expression"));
it("should transform a classnames call", test(type)("classnames"));
it("should transform a spread assignment", test(type)("jsx-spread"));
it("should transform binary expressions", test(type)("binary-expression"));
it("should transform logical expressions", test(type)("logical-expression"));
if (type === "createElement") {
it("should transform prop identifiers", test(type)("identifier-props"));
}
});
});
});
| {'content_hash': '43076315cb7143fc2411d0663d56f90f', 'timestamp': '', 'source': 'github', 'line_count': 44, 'max_line_length': 96, 'avg_line_length': 39.09090909090909, 'alnum_prop': 0.6406976744186047, 'repo_name': 'walmartreact/babel-plugin-react-cssmoduleify', 'id': 'bad341ae7ac5980c6dba6e707386b5683be5f84f', 'size': '1720', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'test/index.js', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'JavaScript', 'bytes': '16208'}]} |
import messages from 'ringcentral-integration/modules/Conference/messages';
export default {
[messages.requireAditionalNumbers]: 'Please select the additional dial-in numbers.'
};
| {'content_hash': 'f7dec885d192dc0d4ca169853898365d', 'timestamp': '', 'source': 'github', 'line_count': 5, 'max_line_length': 85, 'avg_line_length': 36.6, 'alnum_prop': 0.8032786885245902, 'repo_name': 'u9520107/ringcentral-js-widget', 'id': '796b798d783702c4fc56c97555c608c47c939e82', 'size': '183', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'packages/ringcentral-widgets/components/ConferenceAlert/i18n/en-US.js', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '90533'}, {'name': 'HTML', 'bytes': '2967'}, {'name': 'JavaScript', 'bytes': '433434'}, {'name': 'Shell', 'bytes': '1001'}]} |
require File.dirname(__FILE__) + '/../test_helper'
class SalesforceObjectTypeTest < ActiveSupport::TestCase
# Replace this with your real tests.
def test_truth
assert true
end
end
| {'content_hash': '73f773a852484d2421cf5c8a0b65a85b', 'timestamp': '', 'source': 'github', 'line_count': 8, 'max_line_length': 56, 'avg_line_length': 23.875, 'alnum_prop': 0.7225130890052356, 'repo_name': 'raygao/SFRWatcher', 'id': '33f2d2d664eacfc5ea91db62813e6680a0baa629', 'size': '191', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'test/unit/salesforce_object_type_test.rb', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'JavaScript', 'bytes': '85591'}, {'name': 'Ruby', 'bytes': '197148'}]} |
> [Introduced](https://gitlab.com/gitlab-org/gitlab-ee/merge_requests/3507)
in [GitLab Premium](https://about.gitlab.com/pricing/) 10.3.
## Overview
If your application offers a web interface and you are using
[GitLab CI/CD](../../../ci/README.md), you can quickly determine the performance
impact of pending code changes.
GitLab uses [Sitespeed.io](https://www.sitespeed.io), a free and open source
tool for measuring the performance of web sites, and has built a simple
[Sitespeed plugin](https://gitlab.com/gitlab-org/gl-performance)
which outputs the results in a file called `performance.json`. This plugin
outputs the performance score for each page that is analyzed.
The [Sitespeed.io performance score](http://examples.sitespeed.io/6.0/2017-11-23-23-43-35/help.html)
is a composite value based on best practices, and we will be expanding support
for [additional metrics](https://gitlab.com/gitlab-org/gitlab-ee/issues/4370)
in a future release.
Going a step further, GitLab can show the Performance report right
in the merge request widget area:
## Use cases
For instance, consider the following workflow:
1. A member of the marketing team is attempting to track engagement by adding a new tool
1. With browser performance metrics, they see how their changes are impacting the usability of the page for end users
1. The metrics show that after their changes the performance score of the page has gone down
1. When looking at the detailed report, they see that the new Javascript library was included in `<head>` which affects loading page speed
1. They ask a front end developer to help them, who sets the library to load asynchronously
1. The frontend developer approves the merge request and authorizes its deployment to production
## How it works
First of all, you need to define a job in your `.gitlab-ci.yml` file that generates the
[Performance report artifact](../../../ci/yaml/README.md#artifactsreportsperformance-premium).
For more information on how the Performance job should look like, check the
example on [Testing Browser Performance](../../../ci/examples/browser_performance.md).
GitLab then checks this report, compares key performance metrics for each page
between the source and target branches, and shows the information right on the merge request.
>**Note:**
If the Performance report doesn't have anything to compare to, no information
will be displayed in the merge request area. That is the case when you add the
Performance job in your `.gitlab-ci.yml` for the very first time.
Consecutive merge requests will have something to compare to and the Performance
report will be shown properly.

| {'content_hash': 'b6b8e0a4c46e5a4c35211cdc3dc31ec1', 'timestamp': '', 'source': 'github', 'line_count': 52, 'max_line_length': 138, 'avg_line_length': 51.82692307692308, 'alnum_prop': 0.7844155844155845, 'repo_name': 'iiet/iiet-git', 'id': '65ee2e128aed1e530f620d051413214ee8528e60', 'size': '2740', 'binary': False, 'copies': '1', 'ref': 'refs/heads/release', 'path': 'doc/user/project/merge_requests/browser_performance_testing.md', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '694819'}, {'name': 'Clojure', 'bytes': '79'}, {'name': 'Dockerfile', 'bytes': '1907'}, {'name': 'HTML', 'bytes': '1386003'}, {'name': 'JavaScript', 'bytes': '4784137'}, {'name': 'Ruby', 'bytes': '21288676'}, {'name': 'Shell', 'bytes': '47962'}, {'name': 'Vue', 'bytes': '1163492'}]} |
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>ch.qos.logback</groupId>
<artifactId>audit-parent</artifactId>
<version>0.6</version>
<packaging>pom</packaging>
<name>Logback Audit Parent</name>
<description>Logback Audit project</description>
<url>http://audit.qos.ch</url>
<modules>
<module>audit-common</module>
<module>audit-server</module>
<module>audit-client</module>
<module>audit-examples</module>
<module>audit-site</module>
</modules>
<licenses>
<license>
<name>Eclipse Public License - v 1.0</name>
<url>http://www.eclipse.org/legal/epl-v10.html</url>
</license>
<license>
<name>GNU Lesser General Public License</name>
<url>http://www.gnu.org/licenses/old-licenses/lgpl-2.1.html</url>
</license>
</licenses>
<scm>
<url>https://github.com/qos/logback</url>
<connection>[email protected]:qos-ch/logback-audit.git</connection>
</scm>
<developers>
<developer>
<id>ceki</id>
<name>Ceki Gulcu</name>
<email>[email protected]</email>
</developer>
</developers>
<properties>
<slf4j.version>1.7.2</slf4j.version>
<logback.version>1.0.9</logback.version>
<janino.version>2.6.1</janino.version>
</properties>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
</dependencies>
<dependencyManagement>
<dependencies>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>servlet-api</artifactId>
<version>2.5</version>
</dependency>
<dependency>
<groupId>ch.qos.logback</groupId>
<artifactId>audit-common</artifactId>
<version>${project.version}</version>
</dependency>
<dependency>
<groupId>ch.qos.logback</groupId>
<artifactId>audit-client</artifactId>
<version>${project.version}</version>
</dependency>
<dependency>
<groupId>ch.qos.logback</groupId>
<artifactId>logback-core</artifactId>
<version>${logback.version}</version>
</dependency>
<dependency>
<groupId>ch.qos.logback</groupId>
<artifactId>logback-classic</artifactId>
<version>${logback.version}</version>
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>${slf4j.version}</version>
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>jcl-over-slf4j</artifactId>
<version>${slf4j.version}</version>
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-simple</artifactId>
<version>${slf4j.version}</version>
</dependency>
<dependency>
<groupId>janino</groupId>
<artifactId>janino</artifactId>
<version>2.5.10</version>
</dependency>
<dependency>
<groupId>javax.mail</groupId>
<artifactId>mail</artifactId>
<version>1.4</version>
</dependency>
<dependency>
<groupId>dom4j</groupId>
<artifactId>dom4j</artifactId>
<version>1.6.1</version>
</dependency>
<dependency>
<groupId>hsqldb</groupId>
<artifactId>hsqldb</artifactId>
<version>1.8.0.7</version>
</dependency>
</dependencies>
</dependencyManagement>
<build>
<extensions>
<extension>
<groupId>org.apache.maven.wagon</groupId>
<artifactId>wagon-ssh</artifactId>
<version>2.0</version>
</extension>
</extensions>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>2.3.2</version>
<configuration>
<source>1.7</source>
<target>1.7</target>
</configuration>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>2.6</version>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-source-plugin</artifactId>
<version>2.1.2</version>
<executions>
<execution>
<phase>package</phase>
<goals>
<goal>jar</goal>
</goals>
</execution>
</executions>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-eclipse-plugin</artifactId>
<version>2.8</version>
<configuration>
<downloadSources>true</downloadSources>
</configuration>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-assembly-plugin</artifactId>
<version>2.1</version>
<configuration>
<descriptors>
<descriptor>
src/main/assembly/dist.xml
</descriptor>
</descriptors>
<finalName>
logback-audit-${project.version}
</finalName>
<appendAssemblyId>false</appendAssemblyId>
<outputDirectory>target/site/dist/</outputDirectory>
</configuration>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-site-plugin</artifactId>
<version>3.0</version>
<configuration>
<reportPlugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-javadoc-plugin</artifactId>
<version>2.8</version>
<configuration>
<aggregate>true</aggregate>
<links>
<link>
http://java.sun.com/j2se/1.5.0/docs/api
</link>
</links>
<groups>
<group>
<title>Logback Audit Common</title>
<packages>ch.qos.logback.audit</packages>
</group>
<group>
<title>Logback Audit Client</title>
<packages>
ch.qos.logback.audit.client:ch.qos.logback.audit.client.*
</packages>
</group>
<group>
<title>Logback Audit Server</title>
<packages>
ch.qos.logback.audit.server:ch.qos.logback.audit.server.*
</packages>
</group>
<group>
<title>Examples</title>
<packages>examples*</packages>
</group>
</groups>
</configuration>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-jxr-plugin</artifactId>
<version>2.3</version>
<configuration>
<includes>
<include>examples/*.java</include>
</includes>
</configuration>
</plugin>
</reportPlugins>
</configuration>
</plugin>
</plugins>
</build>
<!--<reporting>-->
<!--<plugins>-->
<!--<plugin>-->
<!--<groupId>org.apache.maven.plugins</groupId>-->
<!--<artifactId>maven-project-info-reports-plugin</artifactId>-->
<!--<version>2.4</version>-->
<!--<reportSets>-->
<!--<reportSet><reports/></reportSet>-->
<!--</reportSets>-->
<!--</plugin>-->
<!--</plugins>-->
<!--</reporting>-->
<profiles>
<profile>
<id>license</id>
<build>
<plugins>
<plugin>
<groupId>com.google.code.maven-license-plugin</groupId>
<artifactId>maven-license-plugin</artifactId>
<configuration>
<header>src/main/licenseHeader.txt</header>
<quiet>false</quiet>
<failIfMissing>true</failIfMissing>
<aggregate>true</aggregate>
<includes>
<include>src/**/*.java</include>
</includes>
<useDefaultExcludes>true</useDefaultExcludes>
<useDefaultMapping>true</useDefaultMapping>
<properties>
<year>2006</year>
</properties>
<headerDefinitions>
<headerDefinition>src/main/javadocHeaders.xml</headerDefinition>
</headerDefinitions>
</configuration>
</plugin>
</plugins>
</build>
</profile>
<profile>
<id>javadocjar</id>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-javadoc-plugin</artifactId>
<version>2.8</version>
<executions>
<execution>
<id>attach-javadocs</id>
<goals>
<goal>jar</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
</profile>
<profile>
<id>sign-artifacts</id>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-gpg-plugin</artifactId>
<version>1.1</version>
<executions>
<execution>
<id>sign-artifacts</id>
<phase>verify</phase>
<goals>
<goal>sign</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
</profile>
</profiles>
<distributionManagement>
<site>
<id>pixie</id>
<url>scp://pixie.qos.ch/var/www/audit.qos.ch/htdocs/</url>
</site>
<repository>
<id>sonatype-nexus-staging</id>
<url>https://oss.sonatype.org/service/local/staging/deploy/maven2/</url>
</repository>
</distributionManagement>
</project>
| {'content_hash': '8891614e63ca4aa1d165df9a1d9f045c', 'timestamp': '', 'source': 'github', 'line_count': 368, 'max_line_length': 105, 'avg_line_length': 28.230978260869566, 'alnum_prop': 0.5372028106651265, 'repo_name': 'OBHITA/Consent2Share', 'id': '4df712f17b7f1f2b34f6665c95d8785df7472e6f', 'size': '10389', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'ThirdParty/logback-audit/pom.xml', 'mode': '33188', 'license': 'bsd-3-clause', 'language': [{'name': 'CSS', 'bytes': '491834'}, {'name': 'HTML', 'bytes': '2415468'}, {'name': 'Java', 'bytes': '8076505'}, {'name': 'JavaScript', 'bytes': '659769'}, {'name': 'Ruby', 'bytes': '1504'}, {'name': 'XSLT', 'bytes': '341926'}]} |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>metacoq-safechecker: Not compatible 👼</title>
<link rel="shortcut icon" type="image/png" href="../../../../../favicon.png" />
<link href="../../../../../bootstrap.min.css" rel="stylesheet">
<link href="../../../../../bootstrap-custom.css" rel="stylesheet">
<link href="//maxcdn.bootstrapcdn.com/font-awesome/4.2.0/css/font-awesome.min.css" rel="stylesheet">
<script src="../../../../../moment.min.js"></script>
<!-- HTML5 Shim and Respond.js IE8 support of HTML5 elements and media queries -->
<!-- WARNING: Respond.js doesn't work if you view the page via file:// -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/html5shiv/3.7.2/html5shiv.min.js"></script>
<script src="https://oss.maxcdn.com/respond/1.4.2/respond.min.js"></script>
<![endif]-->
</head>
<body>
<div class="container">
<div class="navbar navbar-default" role="navigation">
<div class="container-fluid">
<div class="navbar-header">
<a class="navbar-brand" href="../../../../.."><i class="fa fa-lg fa-flag-checkered"></i> Coq bench</a>
</div>
<div id="navbar" class="collapse navbar-collapse">
<ul class="nav navbar-nav">
<li><a href="../..">clean / released</a></li>
<li class="active"><a href="">8.15.0 / metacoq-safechecker - 1.0~beta1+8.11</a></li>
</ul>
</div>
</div>
</div>
<div class="article">
<div class="row">
<div class="col-md-12">
<a href="../..">« Up</a>
<h1>
metacoq-safechecker
<small>
1.0~beta1+8.11
<span class="label label-info">Not compatible 👼</span>
</small>
</h1>
<p>📅 <em><script>document.write(moment("2022-04-08 22:17:07 +0000", "YYYY-MM-DD HH:mm:ss Z").fromNow());</script> (2022-04-08 22:17:07 UTC)</em><p>
<h2>Context</h2>
<pre># Packages matching: installed
# Name # Installed # Synopsis
base-bigarray base
base-threads base
base-unix base
conf-findutils 1 Virtual package relying on findutils
conf-gmp 4 Virtual package relying on a GMP lib system installation
coq 8.15.0 Formal proof management system
dune 3.0.3 Fast, portable, and opinionated build system
ocaml 4.10.2 The OCaml compiler (virtual package)
ocaml-base-compiler 4.10.2 Official release 4.10.2
ocaml-config 1 OCaml Switch Configuration
ocamlfind 1.9.3 A library manager for OCaml
zarith 1.12 Implements arithmetic and logical operations over arbitrary-precision integers
# opam file:
opam-version: "2.0"
maintainer: "[email protected]"
homepage: "https://metacoq.github.io/metacoq"
dev-repo: "git+https://github.com/MetaCoq/metacoq.git#coq-8.11"
bug-reports: "https://github.com/MetaCoq/metacoq/issues"
authors: ["Abhishek Anand <[email protected]>"
"Simon Boulier <[email protected]>"
"Cyril Cohen <[email protected]>"
"Yannick Forster <[email protected]>"
"Fabian Kunze <[email protected]>"
"Gregory Malecha <[email protected]>"
"Matthieu Sozeau <[email protected]>"
"Nicolas Tabareau <[email protected]>"
"Théo Winterhalter <[email protected]>"
]
license: "MIT"
build: [
["sh" "./configure.sh"]
[make "-j%{jobs}%" "-C" "safechecker"]
]
install: [
[make "-C" "safechecker" "install"]
]
depends: [
"ocaml" {>= "4.07.1"}
"coq" {>= "8.11" & < "8.12~"}
"coq-metacoq-template" {= version}
"coq-metacoq-checker" {= version}
"coq-metacoq-pcuic" {= version}
]
synopsis: "Implementation and verification of safe conversion and typechecking algorithms for Coq"
description: """
MetaCoq is a meta-programming framework for Coq.
The SafeChecker modules provides a correct implementation of
weak-head reduction, conversion and typechecking of Coq definitions and global environments.
"""
url {
src: "https://github.com/MetaCoq/metacoq/archive/v1.0-beta1-8.11.tar.gz"
checksum: "sha256=1644c5bd9d02385c802535c6c46dbcaf279afcecd4ffb3da5fae08618c628c75"
}
</pre>
<h2>Lint</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Dry install 🏜️</h2>
<p>Dry install with the current Coq version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam install -y --show-action coq-metacoq-safechecker.1.0~beta1+8.11 coq.8.15.0</code></dd>
<dt>Return code</dt>
<dd>5120</dd>
<dt>Output</dt>
<dd><pre>[NOTE] Package coq is already installed (current version is 8.15.0).
The following dependencies couldn't be met:
- coq-metacoq-safechecker -> coq-metacoq-checker = 1.0~beta1+8.11 -> coq < 8.12~ -> ocaml < 4.10
base of this switch (use `--unlock-base' to force)
Your request can't be satisfied:
- No available version of coq satisfies the constraints
No solution found, exiting
</pre></dd>
</dl>
<p>Dry install without Coq/switch base, to test if the problem was incompatibility with the current Coq/OCaml version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam remove -y coq; opam install -y --show-action --unlock-base coq-metacoq-safechecker.1.0~beta1+8.11</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Install dependencies</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>0 s</dd>
</dl>
<h2>Install 🚀</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>0 s</dd>
</dl>
<h2>Installation size</h2>
<p>No files were installed.</p>
<h2>Uninstall 🧹</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Missing removes</dt>
<dd>
none
</dd>
<dt>Wrong removes</dt>
<dd>
none
</dd>
</dl>
</div>
</div>
</div>
<hr/>
<div class="footer">
<p class="text-center">
Sources are on <a href="https://github.com/coq-bench">GitHub</a> © Guillaume Claret 🐣
</p>
</div>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
<script src="../../../../../bootstrap.min.js"></script>
</body>
</html>
| {'content_hash': '99e866bf91e7a53fc00c807dd3ebfe89', 'timestamp': '', 'source': 'github', 'line_count': 182, 'max_line_length': 159, 'avg_line_length': 43.3021978021978, 'alnum_prop': 0.5617307448293364, 'repo_name': 'coq-bench/coq-bench.github.io', 'id': '6b4f8ee1d48037b079bcc5fd6fbfc22a751013f7', 'size': '7907', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'clean/Linux-x86_64-4.10.2-2.0.6/released/8.15.0/metacoq-safechecker/1.0~beta1+8.11.html', 'mode': '33188', 'license': 'mit', 'language': []} |
namespace content {
class RenderProcessHost;
}
#if defined(USE_WEBUI_FILE_PICKER)
namespace wm {
class WMState;
}
#endif
namespace devtools_http_handler {
class DevToolsHttpHandler;
}
namespace xwalk {
class XWalkRunner;
namespace extensions {
class XWalkExtensionService;
}
class XWalkBrowserMainParts : public content::BrowserMainParts {
public:
explicit XWalkBrowserMainParts(
const content::MainFunctionParams& parameters);
~XWalkBrowserMainParts() override;
// BrowserMainParts overrides.
void PreEarlyInitialization() override;
int PreCreateThreads() override;
void PreMainMessageLoopStart() override;
void PostMainMessageLoopStart() override;
void PreMainMessageLoopRun() override;
bool MainMessageLoopRun(int* result_code) override;
void PostMainMessageLoopRun() override;
// Create all the extensions to be hooked into a new
// RenderProcessHost. Base class implementation should be called by
// subclasses overriding this..
virtual void CreateInternalExtensionsForUIThread(
content::RenderProcessHost* host,
extensions::XWalkExtensionVector* extensions);
virtual void CreateInternalExtensionsForExtensionThread(
content::RenderProcessHost* host,
extensions::XWalkExtensionVector* extensions);
devtools_http_handler::DevToolsHttpHandler* devtools_http_handler() {
return devtools_http_handler_.get();
}
protected:
void RegisterExternalExtensions();
XWalkRunner* xwalk_runner_;
extensions::XWalkExtensionService* extension_service_;
// Should be about:blank If no URL is specified in command line arguments.
GURL startup_url_;
// The main function parameters passed to BrowserMain.
const content::MainFunctionParams& parameters_;
// True if we need to run the default message loop defined in content.
bool run_default_message_loop_;
scoped_ptr<devtools_http_handler::DevToolsHttpHandler> devtools_http_handler_;
private:
#if defined(USE_WEBUI_FILE_PICKER)
scoped_ptr<wm::WMState> wm_state_;
#endif
DISALLOW_COPY_AND_ASSIGN(XWalkBrowserMainParts);
};
} // namespace xwalk
#endif // XWALK_RUNTIME_BROWSER_XWALK_BROWSER_MAIN_PARTS_H_
| {'content_hash': 'f97edd8d5bfbdee732e637eed29f6441', 'timestamp': '', 'source': 'github', 'line_count': 80, 'max_line_length': 80, 'avg_line_length': 26.95, 'alnum_prop': 0.7704081632653061, 'repo_name': 'minggangw/crosswalk', 'id': '9a501cf103cd7acce1ef11eec9aa82ea09c13a64', 'size': '2888', 'binary': False, 'copies': '4', 'ref': 'refs/heads/master', 'path': 'runtime/browser/xwalk_browser_main_parts.h', 'mode': '33188', 'license': 'bsd-3-clause', 'language': [{'name': 'C', 'bytes': '36257'}, {'name': 'C#', 'bytes': '10797'}, {'name': 'C++', 'bytes': '1576784'}, {'name': 'CSS', 'bytes': '1709'}, {'name': 'HTML', 'bytes': '118070'}, {'name': 'Java', 'bytes': '1292551'}, {'name': 'JavaScript', 'bytes': '112860'}, {'name': 'Objective-C', 'bytes': '2491'}, {'name': 'Objective-C++', 'bytes': '16628'}, {'name': 'Python', 'bytes': '308084'}, {'name': 'Shell', 'bytes': '2686'}]} |
export class RowMapper {
static map(input: Array<Array<string>>) {
let header = input.shift();
for (let i = 0; i < header.length; i++) {
header[i] = RowMapper.convertToCamelCase(header[i]);
}
let output = [];
for (let row of input) {
let obj = {};
for (let i = 0; i < row.length; i++) {
obj[header[i]] = row[i];
}
output.push(obj);
}
return output;
}
static convertToCamelCase(content: string): string {
// content = content.charAt(0).toLowerCase() + content.slice(1);
// content = contennt.replace(/\s/g, '');
// return content.toLowerCase();
return content.replace(/[^a-z ]/ig, "").replace(/(?:^\w|[A-Z]|\b\w|\s+)/g, function (match, index) {
if (+match === 0) return ""; // or if (/\s+/.test(match)) for white spaces
return index === 0 ? match.toLowerCase() : match.toUpperCase();
});
}
} | {'content_hash': '30858a8057443ce0d00e04c03c114621', 'timestamp': '', 'source': 'github', 'line_count': 34, 'max_line_length': 108, 'avg_line_length': 29.735294117647058, 'alnum_prop': 0.49357072205736896, 'repo_name': 'Savjee/google-sheets-wrapper', 'id': 'dfec3b657d5c3dad97ee17066f4152aed5e9d274', 'size': '1011', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/RowMapper.ts', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'TypeScript', 'bytes': '8334'}]} |
- [`latest`, `LTS`, `LTS-2016`, `8`, `8.10`, `FT` (*8.10/Dockerfile*)](https://github.com/nuxeo/docker-nuxeo/blob/4c45ac6f74a5571dd23ee86a00fb85aadbece05c/8.10/Dockerfile)
- [`8.3` (*8.3/Dockerfile*)](https://github.com/nuxeo/docker-nuxeo/blob/d7252d23d06e0ade25a0054a7ae940e8537a99b2/8.3/Dockerfile)
- [`8.2` (*8.2/Dockerfile*)](https://github.com/nuxeo/docker-nuxeo/blob/a02183111045dadb357e68e11e93c5551c570c43/8.2/Dockerfile)
- [`LTS-2015`, `7`, `7.10` (*7.10/Dockerfile*)](https://github.com/nuxeo/docker-nuxeo/blob/a02183111045dadb357e68e11e93c5551c570c43/7.10/Dockerfile)
- [`LTS-2014`, `6`, `6.0` (*6.0/Dockerfile*)](https://github.com/nuxeo/docker-nuxeo/blob/8535464e476b39e10001242ad7c885519fadc2a6/6.0/Dockerfile)
For more information about this image and its history, please see [the relevant manifest file (`library/nuxeo`)](https://github.com/docker-library/official-images/blob/master/library/nuxeo). This image is updated via [pull requests to the `docker-library/official-images` GitHub repo](https://github.com/docker-library/official-images/pulls?q=label%3Alibrary%2Fnuxeo).
For detailed information about the virtual/transfer sizes and individual layers of each of the above supported tags, please see [the `repos/nuxeo/tag-details.md` file](https://github.com/docker-library/repo-info/blob/master/repos/nuxeo/tag-details.md) in [the `docker-library/repo-info` GitHub repo](https://github.com/docker-library/repo-info).
# What is Nuxeo ?
The Nuxeo Platform is a highly customizable and extensible content management platform for building business applications.
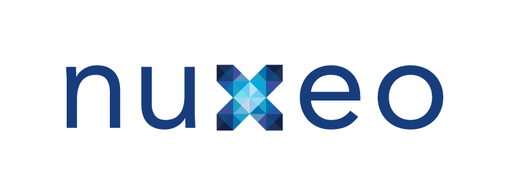
# How to use this image
## Start a bare nuxeo instance
```console
$ docker run --name mynuxeo -p 8080:8080 -d nuxeo
```
This image includes `EXPOSE 8080` (the nuxeo port). The default Nuxeo configuration is applied which feature an embedded database (H2), and an embedded Elasticsearch instance. This setup is not suitable for production. See below to know how to setup a production ready container by specifying environment variables.
The Nuxeo platform is accesible at http://${DOCKER_HOST}:8080/ and default User and Password are Administrator/Administrator.
## Start a nuxeo with some additional packages
```console
$ docker run --name mynuxeo -p 8080:8080 -e NUXEO_PACKAGES="nuxeo-web-mobile nuxeo-drive nuxeo-diff nuxeo-spreadsheet nuxeo-dam nuxeo-template-rendering nuxeo-template-rendering-samples nuxeo-showcase-content"
nuxeo
```
This will install the same image as above but comes with some demo Nuxeo packages to demonstrate its various usage (DAM, DM, search etc...)
## Environment Variables
The Nuxeo image uses several environment variable that allow to specify a more specific setup.
### `NUXEO_DB_TYPE`
This defines the database type to use. By default it sets an H2 embedded database that is suitable for test purpose only. When specifying a DB type, other variable mays help :
- `NUXEO_DB_HOST` : If `NUXEO_DB_TYPE` is defined, this variable is mandatory and has to point to the DB server host.
- `NUXEO_DB_NAME` : name of the database to use (`nuxeo` by default)
- `NUXEO_DB_USER` : user to connect to the database (`nuxeo` by default)
- `NUXEO_DB_PASSWORD` : the password to connect to the database (`nuxeo` by default)
### `NUXEO_TEMPLATES`
This variables allows to add additional [Nuxeo configuration templates](https://doc.nuxeo.com/x/0AB9) in the `nuxeo.templates` configuration variable.
### `NUXEO_ES_HOSTS`
This variables allows to setup an external Elasticsearch cluster. Use a comma separated list of Elasticsearch hosts with the 9300 port. Additional environment vars may be setup like :
- `NUXEO_ES_CLUSTER_NAME` : name of the Elasticsearch cluster to join
- `NUXEO_ES_INDEX_NAME`: name of the index (`nuxeo` by default)
- `NUXEO_ES_REPLICAS` : number or replicas (`1` by default). If not 0, it means that your ES cluster must have enough node to fullfill the replicas setup.
- `NUXEO_ES_SHARDS` : number or shards (`5` by default).
For instance :
NUXEO_ES_HOSTS=es1:9300,es2:9300
NUXEO_ES_CLUSTER_NAME=dockerCluster
NUXEO_ES_INDEX_NAME=nuxeo1
NUXEO_ES_REPLICAS=0
NUXEO_ES_SHARDS=5
### `NUXEO_REDIS_HOST`
In order to use Redis, just set up this variable to the Redis host address.
### `NUXEO_REDIS_PORT`
If Redis is setup, you can ovewrite the default port configuration (default to 6379)
### `NUXEO_CLID`
Allow to setup a CLID for Nuxeo Connect registration.
### `NUXEO_INSTALL_HOTFIX`
This launch the install of latest Hotfixes. (`true` by default, but needs a `NUXEO_CLID` to be setup)
### `NUXEO_PACKAGES`
Allows to install [Nuxeo packages](https://doc.nuxeo.com/x/aAfF) at startup.
### `NUXEO_URL`
This variable sets the URL where your Nuxeo instance will be joinable. It's used for instance to refer to it when sending server's address in mails.
### `NUXEO_DATA`
Location of the Nuxeo data directory. (`/var/lib/nuxeo/data` by default). You will likely customize NUXEO_DATA to map it on docker volume so data like binaries stay persistent.
### `NUXEO_LOG`
Location of the Nuxeo log directory. (`/var/log/nuxeo` by default)
### `NUXEO_AUTOMATION_TRACE`
If set to "true", this will enable the [automation trace mode](https://doc.nuxeo.com/display/NXDOC/Automation+Tracing).
### `NUXEO_DEV_MODE`
If set to "true", this will enable the development mode that will allow [hot reload](https://doc.nuxeo.com/display/CORG/Supporting+Hot+Reload) when developing with [Nuxeo Studio](http://www.nuxeo.com/products/studio/).
### `NUXEO_BINARY_STORE`
Tells the location of the binary store which configure the [binary storage](https://doc.nuxeo.com/x/fYYZAQ)
### `NUXEO_TRANSIENT_STORE`
Tells the location of [the transient storage](http://doc.nuxeo.com/display/NXDOC/Transient+Store)
### `NUXEO_DDL_MODE`
Allows to setup [Database creation option](https://doc.nuxeo.com/x/hwQz#RepositoryConfiguration-DatabaseCreationOption) by fixing the `ddlMode` value.
### `NUXEO_CUSTOM_PARAM`
Allows to add custom parameters to `nuxeo.conf`. Multiple parameters can be splitted by a `\n`. For instance :
NUXEO_CUSTOM_PARAM="repository.clustering.enabled=false\nrepository.clustering.delay=1000"
# How to extend this image
## Adding additional configuration
If you would like to do additional setup in an image derived from this one, you can add a `/nuxeo.conf` file that will be appended to the end of the regular `nuxeo.conf` file.
```dockerfile
FROM nuxeo:7.10
ADD nuxeo.conf /nuxeo.conf
```
## Launching custom shell scripts
You can add your own shell scripts in a special `/docker-entrypoint-initnuxeo.d` directory. When ending in `.sh`, they will be run on default entrypoint startup.
## ffmpeg
As it contains some non-free Codecs, we dont't ship a binary version of `ffmpeg` as part of this image. However, you can simply add the compilation in a derived images by adding these lines to your Dockerfile
```dockerfile
FROM nuxeo:7.10
RUN echo "deb http://httpredir.debian.org/debian jessie non-free" >> /etc/apt/sources.list
RUN apt-get update && apt-get install -y --no-install-recommends libfaac-dev git
WORKDIR /tmp
# Build ffmpeg
ENV BUILD_YASM true
ENV LIBFAAC true
RUN git clone https://github.com/nuxeo/ffmpeg-nuxeo.git
WORKDIR ffmpeg-nuxeo
RUN ./prepare-packages.sh \
&& ./build-yasm.sh \
&& ./build-x264.sh \
&& ./build-libvpx.sh \
&& ./build-ffmpeg.sh \
&& cd /tmp \
&& rm -Rf ffmpeg-nuxeo \
&& rm -rf /var/lib/apt/lists/*
```
## Using Oracle JVM
For the same reasons as `ffmpeg` we don't ship the Oracle JVM and rely by default on OpenJDK. If you want to use the Hotspot JVM you cans add the following lines in a derived Dockerfile
```dockerfile
RUN apt-get remove -y --purge openjdk-8-jdk \
&& add-apt-repository -y ppa:webupd8team/java && apt-get update \
&& echo "debconf shared/accepted-oracle-license-v1-1 select true" | debconf-set-selections \
&& echo "debconf shared/accepted-oracle-license-v1-1 seen true" | debconf-set-selections \
&& apt-get install -y oracle-java8-installer \
&& rm -rf /var/lib/apt/lists/*
```
## Why is this images so big ?
This image is big because it contains a lot of features. The nuxeo distribution itself is about 250M and in order to make cool things work like generating thumbnails or converting document to PDF we need some additional tools that are bundled in the image. We hope that in the future we will be able to delegate those conversions to external services that would be bundled as additional docker images.
# License
View [license information](http://doc.nuxeo.com/x/gIK7) for the software contained in this image.
# Supported Docker versions
This image is officially supported on Docker version 1.12.5.
Support for older versions (down to 1.6) is provided on a best-effort basis.
Please see [the Docker installation documentation](https://docs.docker.com/installation/) for details on how to upgrade your Docker daemon.
# User Feedback
## Issues
If you have any problems with or questions about this image, please contact us through a [GitHub issue](https://github.com/nuxeo/docker-nuxeo/issues). If the issue is related to a CVE, please check for [a `cve-tracker` issue on the `official-images` repository first](https://github.com/docker-library/official-images/issues?q=label%3Acve-tracker).
You can also reach many of the official image maintainers via the `#docker-library` IRC channel on [Freenode](https://freenode.net).
## Contributing
You are invited to contribute new features, fixes, or updates, large or small; we are always thrilled to receive pull requests, and do our best to process them as fast as we can.
Before you start to code, we recommend discussing your plans through a [GitHub issue](https://github.com/nuxeo/docker-nuxeo/issues), especially for more ambitious contributions. This gives other contributors a chance to point you in the right direction, give you feedback on your design, and help you find out if someone else is working on the same thing.
## Documentation
Documentation for this image is stored in the [`nuxeo/` directory](https://github.com/docker-library/docs/tree/master/nuxeo) of the [`docker-library/docs` GitHub repo](https://github.com/docker-library/docs). Be sure to familiarize yourself with the [repository's `README.md` file](https://github.com/docker-library/docs/blob/master/README.md) before attempting a pull request.
| {'content_hash': '65ae485aa966e1ca47867e4b3859367d', 'timestamp': '', 'source': 'github', 'line_count': 216, 'max_line_length': 401, 'avg_line_length': 48.43518518518518, 'alnum_prop': 0.7599885299177978, 'repo_name': 'pydio/docs', 'id': '608e7a9a11da9e3c237f71c885d5e3121d7ca2e8', 'size': '10514', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'nuxeo/README.md', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Perl', 'bytes': '4533'}, {'name': 'Shell', 'bytes': '8741'}]} |
using System.Reflection;
using System.Runtime.CompilerServices;
using System.Runtime.InteropServices;
// General Information about an assembly is controlled through the following
// set of attributes. Change these attribute values to modify the information
// associated with an assembly.
[assembly: AssemblyTitle("UnitTests")]
[assembly: AssemblyDescription("")]
[assembly: AssemblyConfiguration("")]
[assembly: AssemblyCompany("")]
[assembly: AssemblyProduct("UnitTests")]
[assembly: AssemblyCopyright("Copyright © 2013")]
[assembly: AssemblyTrademark("")]
[assembly: AssemblyCulture("")]
// Setting ComVisible to false makes the types in this assembly not visible
// to COM components. If you need to access a type in this assembly from
// COM, set the ComVisible attribute to true on that type.
[assembly: ComVisible(false)]
// The following GUID is for the ID of the typelib if this project is exposed to COM
[assembly: Guid("9e1ff7a8-0171-46c0-8be4-0537255b488d")]
// Version information for an assembly consists of the following four values:
//
// Major Version
// Minor Version
// Build Number
// Revision
//
// You can specify all the values or you can default the Build and Revision Numbers
// by using the '*' as shown below:
// [assembly: AssemblyVersion("1.0.*")]
[assembly: AssemblyVersion("1.0.0.0")]
[assembly: AssemblyFileVersion("1.0.0.0")]
| {'content_hash': '9e481f543e7bc95fd3f92d08b4701f77', 'timestamp': '', 'source': 'github', 'line_count': 36, 'max_line_length': 84, 'avg_line_length': 38.638888888888886, 'alnum_prop': 0.7440690150970525, 'repo_name': 'ernado-x/X.DynamicData', 'id': 'bb2624844f751a9465378a6fd557dea503fe4187', 'size': '1394', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'UnitTests/Properties/AssemblyInfo.cs', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'ASP', 'bytes': '52784'}, {'name': 'C#', 'bytes': '128933'}, {'name': 'CSS', 'bytes': '172121'}, {'name': 'HTML', 'bytes': '3753'}, {'name': 'JavaScript', 'bytes': '102576'}]} |
package com.salesmanager.core.business.search.model;
public class SearchFacet {
private String name;
private String key;
private long count;
public void setKey(String key) {
this.key = key;
}
public String getKey() {
return key;
}
public void setName(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void setCount(long count) {
this.count = count;
}
public long getCount() {
return count;
};
}
| {'content_hash': '1444729c321b368c0c1e46b979e39df7', 'timestamp': '', 'source': 'github', 'line_count': 27, 'max_line_length': 52, 'avg_line_length': 17.0, 'alnum_prop': 0.6884531590413944, 'repo_name': 'asheshsaraf/ecommerce-simple', 'id': 'a743b07b39da4ac6301750365c88826f62958f73', 'size': '459', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'sm-core/src/main/java/com/salesmanager/core/business/search/model/SearchFacet.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'CSS', 'bytes': '535906'}, {'name': 'Java', 'bytes': '2745790'}, {'name': 'JavaScript', 'bytes': '364940'}]} |
SYNONYM
#### According to
The Catalogue of Life, 3rd January 2011
#### Published in
null
#### Original name
null
### Remarks
null | {'content_hash': 'af23e6bb522baecf170eaf4d7bf1cf32', 'timestamp': '', 'source': 'github', 'line_count': 13, 'max_line_length': 39, 'avg_line_length': 10.23076923076923, 'alnum_prop': 0.6917293233082706, 'repo_name': 'mdoering/backbone', 'id': 'b0dfd22111e36e66da958418ebfb4d1e8ed619ad', 'size': '182', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'life/Plantae/Magnoliophyta/Magnoliopsida/Asterales/Asteraceae/Crepis/Crepis vesicaria/ Syn. Lagoseris taurica/README.md', 'mode': '33188', 'license': 'apache-2.0', 'language': []} |
// Copyright 2014 The Rust Project Developers. See the COPYRIGHT
// file at the top-level directory of this distribution and at
// http://rust-lang.org/COPYRIGHT.
//
// Licensed under the Apache License, Version 2.0 <LICENSE-APACHE or
// http://www.apache.org/licenses/LICENSE-2.0> or the MIT license
// <LICENSE-MIT or http://opensource.org/licenses/MIT>, at your
// option. This file may not be copied, modified, or distributed
// except according to those terms.
//! Trait Resolution. See doc.rs.
pub use self::SelectionError::*;
pub use self::FulfillmentErrorCode::*;
pub use self::Vtable::*;
pub use self::ObligationCauseCode::*;
use middle::mem_categorization::Typer;
use middle::subst;
use middle::ty::{mod, Ty};
use middle::infer::InferCtxt;
use std::rc::Rc;
use std::slice::Items;
use syntax::ast;
use syntax::codemap::{Span, DUMMY_SP};
use util::common::ErrorReported;
pub use self::fulfill::FulfillmentContext;
pub use self::select::SelectionContext;
pub use self::select::SelectionCache;
pub use self::select::{MethodMatchResult, MethodMatched, MethodAmbiguous, MethodDidNotMatch};
pub use self::select::{MethodMatchedData}; // intentionally don't export variants
pub use self::util::supertraits;
pub use self::util::transitive_bounds;
pub use self::util::Supertraits;
pub use self::util::search_trait_and_supertraits_from_bound;
mod coherence;
mod fulfill;
mod select;
mod util;
/// An `Obligation` represents some trait reference (e.g. `int:Eq`) for
/// which the vtable must be found. The process of finding a vtable is
/// called "resolving" the `Obligation`. This process consists of
/// either identifying an `impl` (e.g., `impl Eq for int`) that
/// provides the required vtable, or else finding a bound that is in
/// scope. The eventual result is usually a `Selection` (defined below).
#[deriving(Clone)]
pub struct Obligation<'tcx> {
pub cause: ObligationCause<'tcx>,
pub recursion_depth: uint,
pub trait_ref: Rc<ty::TraitRef<'tcx>>,
}
/// Why did we incur this obligation? Used for error reporting.
#[deriving(Clone)]
pub struct ObligationCause<'tcx> {
pub span: Span,
pub code: ObligationCauseCode<'tcx>
}
impl<'tcx> Copy for ObligationCause<'tcx> {}
#[deriving(Clone)]
pub enum ObligationCauseCode<'tcx> {
/// Not well classified or should be obvious from span.
MiscObligation,
/// In an impl of trait X for type Y, type Y must
/// also implement all supertraits of X.
ItemObligation(ast::DefId),
/// Obligation incurred due to an object cast.
ObjectCastObligation(/* Object type */ Ty<'tcx>),
/// To implement drop, type must be sendable.
DropTrait,
/// Various cases where expressions must be sized/copy/etc:
AssignmentLhsSized, // L = X implies that L is Sized
StructInitializerSized, // S { ... } must be Sized
VariableType(ast::NodeId), // Type of each variable must be Sized
ReturnType, // Return type must be Sized
RepeatVec, // [T,..n] --> T must be Copy
// Captures of variable the given id by a closure (span is the
// span of the closure)
ClosureCapture(ast::NodeId, Span),
// Types of fields (other than the last) in a struct must be sized.
FieldSized,
// Only Sized types can be made into objects
ObjectSized,
}
pub type Obligations<'tcx> = subst::VecPerParamSpace<Obligation<'tcx>>;
impl<'tcx> Copy for ObligationCauseCode<'tcx> {}
pub type Selection<'tcx> = Vtable<'tcx, Obligation<'tcx>>;
#[deriving(Clone,Show)]
pub enum SelectionError<'tcx> {
Unimplemented,
Overflow,
OutputTypeParameterMismatch(Rc<ty::TraitRef<'tcx>>, ty::type_err<'tcx>)
}
pub struct FulfillmentError<'tcx> {
pub obligation: Obligation<'tcx>,
pub code: FulfillmentErrorCode<'tcx>
}
#[deriving(Clone)]
pub enum FulfillmentErrorCode<'tcx> {
CodeSelectionError(SelectionError<'tcx>),
CodeAmbiguity,
}
/// When performing resolution, it is typically the case that there
/// can be one of three outcomes:
///
/// - `Ok(Some(r))`: success occurred with result `r`
/// - `Ok(None)`: could not definitely determine anything, usually due
/// to inconclusive type inference.
/// - `Err(e)`: error `e` occurred
pub type SelectionResult<'tcx, T> = Result<Option<T>, SelectionError<'tcx>>;
/// Given the successful resolution of an obligation, the `Vtable`
/// indicates where the vtable comes from. Note that while we call this
/// a "vtable", it does not necessarily indicate dynamic dispatch at
/// runtime. `Vtable` instances just tell the compiler where to find
/// methods, but in generic code those methods are typically statically
/// dispatched -- only when an object is constructed is a `Vtable`
/// instance reified into an actual vtable.
///
/// For example, the vtable may be tied to a specific impl (case A),
/// or it may be relative to some bound that is in scope (case B).
///
///
/// ```
/// impl<T:Clone> Clone<T> for Option<T> { ... } // Impl_1
/// impl<T:Clone> Clone<T> for Box<T> { ... } // Impl_2
/// impl Clone for int { ... } // Impl_3
///
/// fn foo<T:Clone>(concrete: Option<Box<int>>,
/// param: T,
/// mixed: Option<T>) {
///
/// // Case A: Vtable points at a specific impl. Only possible when
/// // type is concretely known. If the impl itself has bounded
/// // type parameters, Vtable will carry resolutions for those as well:
/// concrete.clone(); // Vtable(Impl_1, [Vtable(Impl_2, [Vtable(Impl_3)])])
///
/// // Case B: Vtable must be provided by caller. This applies when
/// // type is a type parameter.
/// param.clone(); // VtableParam(Oblig_1)
///
/// // Case C: A mix of cases A and B.
/// mixed.clone(); // Vtable(Impl_1, [VtableParam(Oblig_1)])
/// }
/// ```
///
/// ### The type parameter `N`
///
/// See explanation on `VtableImplData`.
#[deriving(Show,Clone)]
pub enum Vtable<'tcx, N> {
/// Vtable identifying a particular impl.
VtableImpl(VtableImplData<'tcx, N>),
/// Successful resolution to an obligation provided by the caller
/// for some type parameter.
VtableParam(VtableParamData<'tcx>),
/// Successful resolution for a builtin trait.
VtableBuiltin(VtableBuiltinData<N>),
/// Vtable automatically generated for an unboxed closure. The def
/// ID is the ID of the closure expression. This is a `VtableImpl`
/// in spirit, but the impl is generated by the compiler and does
/// not appear in the source.
VtableUnboxedClosure(ast::DefId, subst::Substs<'tcx>),
/// Same as above, but for a fn pointer type with the given signature.
VtableFnPointer(ty::Ty<'tcx>),
}
/// Identifies a particular impl in the source, along with a set of
/// substitutions from the impl's type/lifetime parameters. The
/// `nested` vector corresponds to the nested obligations attached to
/// the impl's type parameters.
///
/// The type parameter `N` indicates the type used for "nested
/// obligations" that are required by the impl. During type check, this
/// is `Obligation`, as one might expect. During trans, however, this
/// is `()`, because trans only requires a shallow resolution of an
/// impl, and nested obligations are satisfied later.
#[deriving(Clone)]
pub struct VtableImplData<'tcx, N> {
pub impl_def_id: ast::DefId,
pub substs: subst::Substs<'tcx>,
pub nested: subst::VecPerParamSpace<N>
}
#[deriving(Show,Clone)]
pub struct VtableBuiltinData<N> {
pub nested: subst::VecPerParamSpace<N>
}
/// A vtable provided as a parameter by the caller. For example, in a
/// function like `fn foo<T:Eq>(...)`, if the `eq()` method is invoked
/// on an instance of `T`, the vtable would be of type `VtableParam`.
#[deriving(PartialEq,Eq,Clone)]
pub struct VtableParamData<'tcx> {
// In the above example, this would `Eq`
pub bound: Rc<ty::TraitRef<'tcx>>,
}
/// Matches the self type of the inherent impl `impl_def_id`
/// against `self_ty` and returns the resulting resolution. This
/// routine may modify the surrounding type context (for example,
/// it may unify variables).
pub fn select_inherent_impl<'a,'tcx>(infcx: &InferCtxt<'a,'tcx>,
param_env: &ty::ParameterEnvironment<'tcx>,
typer: &Typer<'tcx>,
cause: ObligationCause<'tcx>,
impl_def_id: ast::DefId,
self_ty: Ty<'tcx>)
-> SelectionResult<'tcx,
VtableImplData<'tcx, Obligation<'tcx>>>
{
// This routine is only suitable for inherent impls. This is
// because it does not attempt to unify the output type parameters
// from the trait ref against the values from the obligation.
// (These things do not apply to inherent impls, for which there
// is no trait ref nor obligation.)
//
// Matching against non-inherent impls should be done with
// `try_resolve_obligation()`.
assert!(ty::impl_trait_ref(infcx.tcx, impl_def_id).is_none());
let mut selcx = select::SelectionContext::new(infcx, param_env, typer);
selcx.select_inherent_impl(impl_def_id, cause, self_ty)
}
/// True if neither the trait nor self type is local. Note that `impl_def_id` must refer to an impl
/// of a trait, not an inherent impl.
pub fn is_orphan_impl(tcx: &ty::ctxt,
impl_def_id: ast::DefId)
-> bool
{
!coherence::impl_is_local(tcx, impl_def_id)
}
/// True if there exist types that satisfy both of the two given impls.
pub fn overlapping_impls(infcx: &InferCtxt,
impl1_def_id: ast::DefId,
impl2_def_id: ast::DefId)
-> bool
{
coherence::impl_can_satisfy(infcx, impl1_def_id, impl2_def_id) &&
coherence::impl_can_satisfy(infcx, impl2_def_id, impl1_def_id)
}
/// Given generic bounds from an impl like:
///
/// impl<A:Foo, B:Bar+Qux> ...
///
/// along with the bindings for the types `A` and `B` (e.g., `<A=A0, B=B0>`), yields a result like
///
/// [[Foo for A0, Bar for B0, Qux for B0], [], []]
///
/// Expects that `generic_bounds` have already been fully substituted, late-bound regions liberated
/// and so forth, so that they are in the same namespace as `type_substs`.
pub fn obligations_for_generics<'tcx>(tcx: &ty::ctxt<'tcx>,
cause: ObligationCause<'tcx>,
generic_bounds: &ty::GenericBounds<'tcx>,
type_substs: &subst::VecPerParamSpace<Ty<'tcx>>)
-> subst::VecPerParamSpace<Obligation<'tcx>>
{
util::obligations_for_generics(tcx, cause, 0, generic_bounds, type_substs)
}
pub fn obligation_for_builtin_bound<'tcx>(tcx: &ty::ctxt<'tcx>,
cause: ObligationCause<'tcx>,
source_ty: Ty<'tcx>,
builtin_bound: ty::BuiltinBound)
-> Result<Obligation<'tcx>, ErrorReported>
{
util::obligation_for_builtin_bound(tcx, cause, builtin_bound, 0, source_ty)
}
impl<'tcx> Obligation<'tcx> {
pub fn new(cause: ObligationCause<'tcx>, trait_ref: Rc<ty::TraitRef<'tcx>>)
-> Obligation<'tcx> {
Obligation { cause: cause,
recursion_depth: 0,
trait_ref: trait_ref }
}
pub fn misc(span: Span, trait_ref: Rc<ty::TraitRef<'tcx>>) -> Obligation<'tcx> {
Obligation::new(ObligationCause::misc(span), trait_ref)
}
pub fn self_ty(&self) -> Ty<'tcx> {
self.trait_ref.self_ty()
}
}
impl<'tcx> ObligationCause<'tcx> {
pub fn new(span: Span, code: ObligationCauseCode<'tcx>)
-> ObligationCause<'tcx> {
ObligationCause { span: span, code: code }
}
pub fn misc(span: Span) -> ObligationCause<'tcx> {
ObligationCause { span: span, code: MiscObligation }
}
pub fn dummy() -> ObligationCause<'tcx> {
ObligationCause { span: DUMMY_SP, code: MiscObligation }
}
}
impl<'tcx, N> Vtable<'tcx, N> {
pub fn iter_nested(&self) -> Items<N> {
match *self {
VtableImpl(ref i) => i.iter_nested(),
VtableFnPointer(..) => (&[]).iter(),
VtableUnboxedClosure(..) => (&[]).iter(),
VtableParam(_) => (&[]).iter(),
VtableBuiltin(ref i) => i.iter_nested(),
}
}
pub fn map_nested<M>(&self, op: |&N| -> M) -> Vtable<'tcx, M> {
match *self {
VtableImpl(ref i) => VtableImpl(i.map_nested(op)),
VtableFnPointer(ref sig) => VtableFnPointer((*sig).clone()),
VtableUnboxedClosure(d, ref s) => VtableUnboxedClosure(d, s.clone()),
VtableParam(ref p) => VtableParam((*p).clone()),
VtableBuiltin(ref b) => VtableBuiltin(b.map_nested(op)),
}
}
pub fn map_move_nested<M>(self, op: |N| -> M) -> Vtable<'tcx, M> {
match self {
VtableImpl(i) => VtableImpl(i.map_move_nested(op)),
VtableFnPointer(sig) => VtableFnPointer(sig),
VtableUnboxedClosure(d, s) => VtableUnboxedClosure(d, s),
VtableParam(p) => VtableParam(p),
VtableBuiltin(no) => VtableBuiltin(no.map_move_nested(op)),
}
}
}
impl<'tcx, N> VtableImplData<'tcx, N> {
pub fn iter_nested(&self) -> Items<N> {
self.nested.iter()
}
pub fn map_nested<M>(&self,
op: |&N| -> M)
-> VtableImplData<'tcx, M>
{
VtableImplData {
impl_def_id: self.impl_def_id,
substs: self.substs.clone(),
nested: self.nested.map(op)
}
}
pub fn map_move_nested<M>(self, op: |N| -> M)
-> VtableImplData<'tcx, M> {
let VtableImplData { impl_def_id, substs, nested } = self;
VtableImplData {
impl_def_id: impl_def_id,
substs: substs,
nested: nested.map_move(op)
}
}
}
impl<N> VtableBuiltinData<N> {
pub fn iter_nested(&self) -> Items<N> {
self.nested.iter()
}
pub fn map_nested<M>(&self,
op: |&N| -> M)
-> VtableBuiltinData<M>
{
VtableBuiltinData {
nested: self.nested.map(op)
}
}
pub fn map_move_nested<M>(self, op: |N| -> M) -> VtableBuiltinData<M> {
VtableBuiltinData {
nested: self.nested.map_move(op)
}
}
}
impl<'tcx> FulfillmentError<'tcx> {
fn new(obligation: Obligation<'tcx>, code: FulfillmentErrorCode<'tcx>)
-> FulfillmentError<'tcx>
{
FulfillmentError { obligation: obligation, code: code }
}
pub fn is_overflow(&self) -> bool {
match self.code {
CodeAmbiguity => false,
CodeSelectionError(Overflow) => true,
CodeSelectionError(_) => false,
}
}
}
| {'content_hash': '7902609885ce81d551403c8b9729fba8', 'timestamp': '', 'source': 'github', 'line_count': 422, 'max_line_length': 99, 'avg_line_length': 35.86492890995261, 'alnum_prop': 0.6104393789230261, 'repo_name': 'emk/rust', 'id': 'd410a456dc913d01e8df8853c13a12daae78e7cd', 'size': '15135', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/librustc/middle/traits/mod.rs', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'ANTLR', 'bytes': '3093'}, {'name': 'Assembly', 'bytes': '25901'}, {'name': 'Awk', 'bytes': '159'}, {'name': 'C', 'bytes': '676528'}, {'name': 'C++', 'bytes': '62321'}, {'name': 'CSS', 'bytes': '21483'}, {'name': 'Emacs Lisp', 'bytes': '43099'}, {'name': 'JavaScript', 'bytes': '32526'}, {'name': 'Makefile', 'bytes': '201910'}, {'name': 'Pascal', 'bytes': '1654'}, {'name': 'Perl', 'bytes': '1076'}, {'name': 'Puppet', 'bytes': '9596'}, {'name': 'Python', 'bytes': '105676'}, {'name': 'Rust', 'bytes': '17189000'}, {'name': 'Shell', 'bytes': '294889'}, {'name': 'VimL', 'bytes': '36290'}]} |
<?php
/**
* This class provides a list of HTTP status codes and their values
*
* @package Nails
* @subpackage common
* @category Library
* @author Nails Dev Team
*/
namespace Nails\Common\Service;
class HttpCodes
{
// 1xx Informational
const STATUS_100 = 'Continue';
const STATUS_CONTINUE = 100;
const STATUS_101 = 'Switching Protocols';
const STATUS_SWITCHING_PROTOCOLS = 101;
// 2xx Success
const STATUS_200 = 'OK';
const STATUS_OK = 200;
const STATUS_201 = 'Created';
const STATUS_CREATED = 201;
const STATUS_202 = 'Accepted';
const STATUS_ACCEPTED = 202;
const STATUS_203 = 'Non-Authoritative Information';
const STATUS_NON_AUTHORITATIVE_INFORMATION = 203;
const STATUS_204 = 'No Content';
const STATUS_NO_CONTENT = 204;
const STATUS_205 = 'Reset Content';
const STATUS_RESET_CONTENT = 205;
const STATUS_206 = 'Partial Content';
const STATUS_PARTIAL_CONTENT = 206;
const STATUS_207 = 'Multi-status';
const STATUS_MULTI_STATUS = 207;
const STATUS_208 = 'Already imported';
const STATUS_ALREADY_IMPORTED = 208;
const STATUS_226 = 'IM used';
const STATUS_IM_USED = 226;
// 3xx Redirection
const STATUS_300 = 'Multiple Choices';
const STATUS_MULTIPLE_CHOICES = 300;
const STATUS_301 = 'Moved Permanently';
const STATUS_MOVED_PERMANENTLY = 301;
const STATUS_302 = 'Found';
const STATUS_FOUND = 302;
const STATUS_303 = 'See Other';
const STATUS_SEE_OTHER = 303;
const STATUS_304 = 'Not Modified';
const STATUS_NOT_MODIFIED = 304;
const STATUS_305 = 'Use Proxy';
const STATUS_USE_PROXY = 305;
const STATUS_306 = 'Switch Proxy';
const STATUS_SWITCH_PROXY = 306;
const STATUS_307 = 'Temporary redirect';
const STATUS_TEMPORARY_REDIRECT = 307;
const STATUS_308 = 'Permanent redirect';
const STATUS_PERMANENT_REDIRECT = 308;
// 4xxx Client Error
const STATUS_400 = 'Bad Request';
const STATUS_BAD_REQUEST = 400;
const STATUS_401 = 'Unauthorized';
const STATUS_UNAUTHORIZED = 401;
const STATUS_402 = 'Payment Required';
const STATUS_PAYMENT_REQUIRED = 402;
const STATUS_403 = 'Forbidden';
const STATUS_FORBIDDEN = 403;
const STATUS_404 = 'Not Found';
const STATUS_NOT_FOUND = 404;
const STATUS_405 = 'Method Not Allowed';
const STATUS_METHOD_NOT_ALLOWED = 405;
const STATUS_406 = 'Not Acceptable';
const STATUS_NOT_ACCEPTABLE = 406;
const STATUS_407 = 'Proxy Authentication Required';
const STATUS_PROXY_AUTHENTICATION_REQUIRED = 407;
const STATUS_408 = 'Request Timeout';
const STATUS_REQUEST_TIMEOUT = 408;
const STATUS_409 = 'Conflict';
const STATUS_CONFLICT = 409;
const STATUS_410 = 'Gone';
const STATUS_GONE = 410;
const STATUS_411 = 'Length Required';
const STATUS_LENGTH_REQUIRED = 411;
const STATUS_412 = 'Precondition Failed';
const STATUS_PRECONDITION_FAILED = 412;
const STATUS_413 = 'Request Entity Too Large';
const STATUS_REQUEST_ENTITY_TOO_LARGE = 413;
const STATUS_414 = 'Request-URI Too Long';
const STATUS_REQUES_URI_TOO_LONG = 414;
const STATUS_415 = 'Unsupported Media Type';
const STATUS_UNSUPPORTED_MEDIA_TYPE = 415;
const STATUS_416 = 'Requested Range Not Satisfiable';
const STATUS_REQUESTED_RANGE_NOT_SATISFIABLE = 416;
const STATUS_417 = 'Expectation Failed';
const STATUS_EXPECTATION_FAILED = 417;
const STATUS_421 = 'Misdirected request';
const STATUS_MISDIRECTED_REQUEST = 421;
const STATUS_422 = 'Unprocessable entity';
const STATUS_UNPROCESSABLE_ENTITY = 422;
const STATUS_423 = 'Locked';
const STATUS_LOCKED = 423;
const STATUS_424 = 'Failed dependency';
const STATUS_FAILED_DEPENDENCY = 424;
const STATUS_426 = 'Upgrade required';
const STATUS_UPGRADE_REQUIRED = 426;
const STATUS_428 = 'Precondition required';
const STATUS_PRECONDITION_REQUIRED = 428;
const STATUS_429 = 'Too many requests';
const STATUS_TOO_MANY_REQUESTS = 429;
const STATUS_431 = 'Request header fields too large';
const STATUS_REQUEST_HEADER_FIELDS_TOO_LARGE = 431;
// 5xx Server Error
const STATUS_500 = 'Internal Server Error';
const STATUS_INTERNAL_SERVER_ERROR = 500;
const STATUS_501 = 'Not Implemented';
const STATUS_NOT_IMPLEMENTED = 501;
const STATUS_502 = 'Bad Gateway';
const STATUS_BAD_GATEWAY = 502;
const STATUS_503 = 'Service Unavailable';
const STATUS_SERVICE_UNAVAILABLE = 503;
const STATUS_504 = 'Gateway Timeout';
const STATUS_GATEWAY_TIMEOUT = 504;
const STATUS_505 = 'HTTP Version Not Supported';
const STATUS_HTTP_VERSION_NOT_SUPPORTED = 505;
const STATUS_506 = 'Variant also negotiates';
const STATUS_VARIANT_ALSO_NEGOTIATES = 506;
const STATUS_507 = 'Insufficient storage';
const STATUS_INSUFFICIENT_STORAGE = 507;
const STATUS_508 = 'Loop detected';
const STATUS_LOOP_DETECTED = 508;
const STATUS_510 = 'Not extended';
const STATUS_NOT_EXTENDED = 510;
const STATUS_511 = 'Network authentication required';
const STATUS_NETWORK_AUTHENTICATION_REQUIRED = 511;
// --------------------------------------------------------------------------
/**
* Returns the human readable portion of an HTTP status code
*
* @param $iCode integer The numerical HTTP status code
*
* @return null
*/
public static function getByCode($iCode)
{
$sConstant = 'static::STATUS_' . $iCode;
if (defined($sConstant)) {
return constant($sConstant);
}
return null;
}
}
| {'content_hash': 'b597f7feca2a35e39b0c3484c9b21c5b', 'timestamp': '', 'source': 'github', 'line_count': 161, 'max_line_length': 85, 'avg_line_length': 48.84472049689441, 'alnum_prop': 0.4763479145473042, 'repo_name': 'nailsapp/common', 'id': 'd5aecb7ece62a97dd3ca4128815a2c61d7f3f296', 'size': '7864', 'binary': False, 'copies': '1', 'ref': 'refs/heads/develop', 'path': 'src/Common/Service/HttpCodes.php', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '8531'}, {'name': 'HTML', 'bytes': '5964'}, {'name': 'Hack', 'bytes': '57'}, {'name': 'JavaScript', 'bytes': '1182'}, {'name': 'PHP', 'bytes': '1032959'}]} |
typedef void(^SFAlertViewCompletion)(NSInteger buttonIndex, NSString *buttonTitle);
@interface UIAlertView (SFAddition_quickAlert)
+ (void)sf_dismissPresentingDialogAnimated:(BOOL)animated __attribute__((deprecated));
+ (UIAlertView *)sf_alertWithTitle:(NSString *)title message:(NSString *)message completion:(SFAlertViewCompletion)completion cancelButtonTitle:(NSString *)cancelButtonTitle otherButtonTitles:(NSString *)otherButtonTitles, ... __attribute__((deprecated));
+ (UIAlertView *)sf_alertWithTitle:(NSString *)title message:(NSString *)message completion:(SFAlertViewCompletion)completion cancelButtonTitle:(NSString *)cancelButtonTitle otherButtonTitleList:(NSArray *)otherButtonTitleList __attribute__((deprecated));
+ (UIAlertView *)sf_alertWithTitle:(NSString *)title message:(NSString *)message completion:(void(^)(void))completion __attribute__((deprecated));
+ (UIAlertView *)sf_alertWithMessage:(NSString *)message completion:(void (^)(void))completion __attribute__((deprecated));
@end
@interface UIAlertView (SFAddition_confirmDialog)
+ (void)sf_confirmWithTitle:(NSString *)title message:(NSString *)message approve:(void(^)(void))approve __attribute__((deprecated));
+ (void)sf_confirmWithTitle:(NSString *)title message:(NSString *)message approve:(void(^)(void))approve cancel:(void(^)(void))cancel __attribute__((deprecated));
@end
@interface UIAlertView (SFAddition_inputDialog)
+ (UITextField *)sf_inputWithTitle:(NSString *)title message:(NSString *)message cancelButtonTitle:(NSString *)cancelButtonTitle approveButtonTitle:(NSString *)approveButtonTitle completion:(void(^)(NSString *input, BOOL cancelled))completion __attribute__((deprecated));
+ (UITextField *)sf_inputWithTitle:(NSString *)title message:(NSString *)message secureTextEntry:(BOOL)secureTextEntry cancelButtonTitle:(NSString *)cancelButtonTitle approveButtonTitle:(NSString *)approveButtonTitle completion:(void(^)(NSString *input, BOOL cancelled))completion __attribute__((deprecated));
@end
@interface UIAlertView (SFAddition)
- (UILabel *)sf_messageLabel __attribute__((deprecated));
- (UILabel *)sf_titleLabel __attribute__((deprecated));
@end
| {'content_hash': '309ab5bf0cf4c8b808c6eeeacb7ab59a', 'timestamp': '', 'source': 'github', 'line_count': 37, 'max_line_length': 309, 'avg_line_length': 58.513513513513516, 'alnum_prop': 0.7778290993071594, 'repo_name': 'yangzexin/SFLibraries', 'id': '51c35ce0ce5ff2971305298ab135dde8424dbdb4', 'size': '2354', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'SFiOSKit/SFiOSKit/UIAlertView+SFAddition.h', 'mode': '33261', 'license': 'apache-2.0', 'language': [{'name': 'JavaScript', 'bytes': '816'}, {'name': 'Objective-C', 'bytes': '592585'}, {'name': 'Ruby', 'bytes': '1810'}]} |
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="rectangle">
<solid android:color="#399C03"/>
<corners android:radius="25dp"/>
</shape> | {'content_hash': 'a4b16a9a0a5370edbf93d4025b098d11', 'timestamp': '', 'source': 'github', 'line_count': 7, 'max_line_length': 65, 'avg_line_length': 31.285714285714285, 'alnum_prop': 0.6757990867579908, 'repo_name': 'mohdaquib/DairyWala', 'id': '535220fa48c3eadc02d300823e8a87a433f5bc9b', 'size': '219', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'app/src/main/res/drawable/drawable_create_account_button.xml', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Java', 'bytes': '235783'}]} |
//## begin module%3744298B0396.cm preserve=no
// %X% %Q% %Z% %W%
//## end module%3744298B0396.cm
//## begin module%3744298B0396.cp preserve=yes
// ********************************************************************************
//
// Copyright: Nortel Networks 1999
//
// Classification: Confidential
//
// Project Name: RRM Simulator / Scheduler
//
// Package: Statistics Calculation
//
// File Name: ContinuousHistogram.cpp
//
// Description: Implementation file for the ContinuousHistogram class.
//
// Author: Hélio Azevedo
//
// Creation Date: May/20/99
//
// Visual Source Safe $Revision: 8 $
//
// Last check in $Date: 16/06/99 9:28 $
//
// ********************************************************************************
//## end module%3744298B0396.cp
//## Module: ContinuousHistogram%3744298B0396; Pseudo Package body
//## Source file: T:\StatisticsCalculation\ContinuousHistogram.cpp
//## begin module%3744298B0396.additionalIncludes preserve=no
//## end module%3744298B0396.additionalIncludes
//## begin module%3744298B0396.includes preserve=yes
#include "MemLeak.h"
#include <assert.h> // define the assert macro
//## end module%3744298B0396.includes
// ContinuousHistogram
#include "ContinuousHistogram.h"
//## begin module%3744298B0396.additionalDeclarations preserve=yes
using namespace std;
namespace sch {
//## end module%3744298B0396.additionalDeclarations
// Class ContinuousHistogram
//## Operation: ContinuousHistogram%37442A26021C
// ********************************************************************************
//
// Name: ContinuousHistogram(Float minVal,Float maxVal, Int intervals)
//
// Description: Non-default constructor - requires the lower boundary, upper boundary and number of intervals. The pValues array is
// allocated.
//
// Input parameters: Float minVal; //lower boundary of the input data stream
// Float maxVal; //upper boundary of the input data stream
// Int intervals; //number of intervals in the histogram
//
// Returns: none
//
// Exceptions: SchException, with one of the following error codes:
// SCH_ALLOCATION_ERROR = could not allocate pValues array
// SCH_INVALID_RANGE = upper boundary is not greater than lower boundary
//
// ********************************************************************************
ContinuousHistogram::ContinuousHistogram (Float minVal, Float maxVal, Int intervals)
//## begin ContinuousHistogram::ContinuousHistogram%37442A26021C.hasinit preserve=no
: maxValue(maxVal),
minValue(minVal),
numIntervals(intervals),
pValues(NULL),
maxSample(SIM_HUGE_VAL),
minSample(SIM_HUGE_VAL),
numSamples(0),
cumSum(0.0),
cumSumOfSquares(0.0)
//## end ContinuousHistogram::ContinuousHistogram%37442A26021C.hasinit
//## begin ContinuousHistogram::ContinuousHistogram%37442A26021C.initialization preserve=yes
//## end ContinuousHistogram::ContinuousHistogram%37442A26021C.initialization
{
//## begin ContinuousHistogram::ContinuousHistogram%37442A26021C.body preserve=yes
RetCode rc = SCH_SUCCESS; // used to provide a single point for raising exception
//
// upper boundary must be greater than lower boundary
//
if (minValue > maxValue)
{
rc = SCH_INVALID_RANGE;
}
if (rc == SCH_SUCCESS)
{
//
// calculate width of each interval
//
intervalWidth = (maxValue - minValue) / numIntervals;
//
// allocate pValues array
//
pValues = new Int[numIntervals]; // allocate array
if (pValues == NULL)
{
rc = SCH_ALLOCATION_ERROR; // could not create array
}
else
{
memset (pValues, 0, numIntervals * sizeof (Int)); // set all values to 0
}
}
if (rc != SCH_SUCCESS)
{
throw SchException (rc); // an error occurred, abort object construction
}
return;
//## end ContinuousHistogram::ContinuousHistogram%37442A26021C.body
}
//## Other Operations (implementation)
//## Operation: getResult%37442A34033F
// ********************************************************************************
//
// Name: RetCode getResult(Int intervalNumber, Int *pResult)
//
// Description: Returns the contents (frequency) of the corresponding interval number.
//
// Input parameters: Int intervalNumber; //first interval number is zero and the last is interval-1
//
// Output parameters: Int *pResult; //contents of the corresponding interval number
//
// Returns: SCH_SUCCESS
// SCH_INVALID_PARAMETER - pResult is NULL
// SCH_INVALID_INTERVAL - interval number is negative or above higher interval - ignore contents of pResult
//
// ********************************************************************************
RetCode ContinuousHistogram::getResult (Int intervalNumber, Int *pResult)
{
//## begin ContinuousHistogram::getResult%37442A34033F.body preserve=yes
RetCode rc = SCH_SUCCESS;
assert (pValues);
if (pResult == NULL)
{
rc = SCH_INVALID_PARAMETER;
}
//
// intervalNumber within valid range?
//
else if ((intervalNumber >= 0) && (intervalNumber < numIntervals))
{
*pResult = pValues[intervalNumber]; // yes, return interval frequency
}
else
{
rc = SCH_INVALID_INTERVAL;
}
return (rc);
//## end ContinuousHistogram::getResult%37442A34033F.body
}
//## Operation: nextSample%37442A34035D
// ********************************************************************************
//
// Name: RetCode nextSample (Float sample)
//
// Description: Includes the next sample in the sequence and increments the contents of the interval which this sample falls into.
//
// Input parameters:Float sample; //next sample in the sequence
//
// Returns: SCH_SUCCESS
//
// ********************************************************************************
RetCode ContinuousHistogram::nextSample (Float sample)
{
//## begin ContinuousHistogram::nextSample%37442A34035D.body preserve=yes
RetCode rc = SCH_SUCCESS;
Int index; // position of pValues to be incremented
assert (pValues);
// update minimum and maximun samples
if (numSamples == 0) {
maxSample = sample;
minSample = sample;
}
else {
if (sample < minSample) minSample = sample;
if (sample > maxSample) maxSample = sample;
}
numSamples++; // increments number of samples
cumSum = cumSum + sample;
cumSumOfSquares = cumSumOfSquares + sample*sample;
if (sample < minValue)
{
//
// account sample in first interval if sample is below the lower boundary
//
index = 0;
}
else if (sample >= maxValue)
{
//
// account sample in last interval if sample is above the higher boundary
//
index = numIntervals - 1;
}
else
{
//
// account sample in appropriate interval
// the interval number is actually the index of pValues, which is calculated by
// index = (sample - minValue) div intervalWidth
// where div is an integer division
//
index = (sample - minValue) / intervalWidth;
}
//
// increment contents of target interval
//
pValues [index]++;
return (rc);
//## end ContinuousHistogram::nextSample%37442A34035D.body
}
//## Operation: reset%374C031B03A1
// ********************************************************************************
//
// Name:RetCode reset (void)
//
// Description: Reset all attributes allowing the start of a new sequence of samples.
//
// Parameters: none
//
// Returns: SCH_SUCCESS
// Error code in case of error
//
// Remarks:Note that all old information is lost after the reset.
//
// ********************************************************************************
RetCode ContinuousHistogram::reset ()
{
//## begin ContinuousHistogram::reset%374C031B03A1.body preserve=yes
// resets attributes variables
maxSample = SIM_HUGE_VAL;
minSample = SIM_HUGE_VAL;
numSamples = 0;
cumSum = 0.0;
cumSumOfSquares = 0.0;
if (pValues == NULL)
return SCH_ALLOCATION_ERROR; // the objetct has not been created
else
memset (pValues, 0, numIntervals * sizeof (Int)); // set all values to 0
return SCH_SUCCESS;
//## end ContinuousHistogram::reset%374C031B03A1.body
}
// Additional Declarations
//## begin ContinuousHistogram%3744298B0396.declarations preserve=yes
//## end ContinuousHistogram%3744298B0396.declarations
//## begin module%3744298B0396.epilog preserve=yes
} // namespace sch
//## end module%3744298B0396.epilog
| {'content_hash': '3497174067e147a9b4a7da53a54e1b8c', 'timestamp': '', 'source': 'github', 'line_count': 311, 'max_line_length': 131, 'avg_line_length': 28.041800643086816, 'alnum_prop': 0.6068111455108359, 'repo_name': 'aclisp/large-scale', 'id': 'c162d854b6f7f636ad10ae130a2bd685d82c127b', 'size': '8898', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'Simulator/Scheduler/StatisticsCalculation/ContinuousHistogram.cpp', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Awk', 'bytes': '913'}, {'name': 'C', 'bytes': '5141993'}, {'name': 'C++', 'bytes': '1756184'}, {'name': 'CSS', 'bytes': '12830'}, {'name': 'Java', 'bytes': '28931'}, {'name': 'Objective-C', 'bytes': '11541'}, {'name': 'Python', 'bytes': '3656'}, {'name': 'Shell', 'bytes': '2157'}]} |
package com.bbva.kltt.apirest.core.generator.output.language;
import org.junit.Test;
import com.bbva.kltt.apirest.core.parsed_info.ParsedInfoHandlerTest;
import com.bbva.kltt.apirest.core.parsed_info.responses.ResponseTest;
import com.bbva.kltt.apirest.core.util.APIRestGeneratorException;
import com.bbva.kltt.apirest.core.util.ConstantsTest;
/**
* ------------------------------------------------
* @author Francisco Manuel Benitez Chico
* ------------------------------------------------
*/
public class OutputLanguageExceptionsTest
{
@Test
public void fullTest() throws APIRestGeneratorException
{
final OutputLanguageExceptions outputLanguageExceptions = new OutputLanguageExceptions(ParsedInfoHandlerTest.generateDummyParsedInfoHandler(),
new MyOutputLanguageNaming(),
new MyOutputLanguageOperations()) ;
outputLanguageExceptions.getCommonException() ;
outputLanguageExceptions.getCommonExceptionClassName() ;
outputLanguageExceptions.getCustomExceptionAsClassName(ConstantsTest.OPERATION_ID, ResponseTest.generateDummyResponse()) ;
outputLanguageExceptions.getCustomExceptionsList(ConstantsTest.OPERATION_ID, ConstantsTest.PATH_OPERATION) ;
outputLanguageExceptions.getOutboundServerExceptionsMap() ;
outputLanguageExceptions.getParsedInfoHandler() ;
}
}
| {'content_hash': 'b1a6692b2ea1b6d37ead704f2e100a0c', 'timestamp': '', 'source': 'github', 'line_count': 32, 'max_line_length': 145, 'avg_line_length': 43.125, 'alnum_prop': 0.7231884057971014, 'repo_name': 'BBVA-CIB/APIRestGenerator', 'id': 'b835b3f51346c17bb56871ac848b9d6f4883e1a4', 'size': '1380', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'core/src/test/java/com/bbva/kltt/apirest/core/generator/output/language/OutputLanguageExceptionsTest.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Batchfile', 'bytes': '229'}, {'name': 'CSS', 'bytes': '504463'}, {'name': 'HTML', 'bytes': '9688'}, {'name': 'Java', 'bytes': '1098093'}, {'name': 'JavaScript', 'bytes': '136674'}, {'name': 'Shell', 'bytes': '253'}]} |
{% extends "theme_bootstrap/base.html" %}
{% load staticfiles %}
{% load metron_tags %}
{% load i18n %}
{% block styles %}
{% include "_styles.html" %}
<style>
html{
width:100%;height:100%;
}
</style>
{% endblock %}
{% block extra_head_base %}
{% block extra_head %}
<script src="{%static 'js/angular.min.js'%}"></script>
<script src="{%static 'js/angular-route.min.js'%}"></script>
<script src="{%static 'js/angular-sanitize.min.js'%}"></script>
<script src="{%static 'js/jquery-1.9.1.min.js'%}"></script>
{% endblock %}
{% endblock %}
{% block topbar_base %}
<header>
<div class="navbar navbar-default {% block navbar_class %}navbar-fixed-top{% endblock %}">
<div class="container">
{% block topbar %}
<div class="navbar-header">
<button class="navbar-toggle" data-toggle="collapse" data-target=".navbar-collapse">
<span class="fa fa-bars"></span>
</button>
{% block site_brand %}<a class="navbar-brand" href="{% url "home" %}">{{ SITE_NAME }}</a>{% endblock %}
</div>
<div class="collapse navbar-collapse navbar-responsive-collapse">
{% block nav %}
<ul class="nav navbar-nav pull-left">
<li><a href="{% url 'profiles_list' %}">People</a></li>
<li><a href="{% url 'team_list' %}">Teams</a></li>
</ul>
{% endblock %}
{% block account_bar %}{% include "_account_bar.html" %}{% endblock %}
</div>
{% endblock %}
</div>
</div>
</header>
{% endblock %}
{% block footer %}
{% include "_footer.html" %}
{% endblock %}
{% block scripts %}
{% include "_scripts.html" %}
<script src="{%static 'bootbox/bootbox.min.js'%}"></script>
<script src="{%static 'js/ui-bootstrap-tpls-0.13.3.min.js'%}"></script>
{% endblock %}
{% block extra_body_base %}
{% analytics %}
{% block extra_body %}{% endblock %}
{% endblock %}
| {'content_hash': '523519c31849312ebe7e416186c6c6a6', 'timestamp': '', 'source': 'github', 'line_count': 67, 'max_line_length': 119, 'avg_line_length': 30.074626865671643, 'alnum_prop': 0.5325062034739454, 'repo_name': 'bird50/birdproj', 'id': 'eb90832f3f0aa69dc8baa17e2780b6ab7a898524', 'size': '2015', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'mapservice/templates/_service_base.html', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '136307'}, {'name': 'HTML', 'bytes': '500185'}, {'name': 'Java', 'bytes': '45492'}, {'name': 'JavaScript', 'bytes': '3734934'}, {'name': 'Makefile', 'bytes': '773'}, {'name': 'PHP', 'bytes': '3138'}, {'name': 'Python', 'bytes': '68448'}, {'name': 'Shell', 'bytes': '410'}]} |
<?php
namespace App\Test\TestCase\Model\Table;
use App\Model\Table\RoomsTable;
use Cake\ORM\TableRegistry;
use Cake\TestSuite\TestCase;
/**
* App\Model\Table\RoomsTable Test Case
*/
class RoomsTableTest extends TestCase
{
/**
* Test subject
*
* @var \App\Model\Table\RoomsTable
*/
public $Rooms;
/**
* Fixtures
*
* @var array
*/
public $fixtures = [
'app.rooms',
'app.statuses'
];
/**
* setUp method
*
* @return void
*/
public function setUp()
{
parent::setUp();
$config = TableRegistry::exists('Rooms') ? [] : ['className' => RoomsTable::class];
$this->Rooms = TableRegistry::get('Rooms', $config);
}
/**
* tearDown method
*
* @return void
*/
public function tearDown()
{
unset($this->Rooms);
parent::tearDown();
}
/**
* Test initialize method
*
* @return void
*/
public function testInitialize()
{
$this->markTestIncomplete('Not implemented yet.');
}
/**
* Test validationDefault method
*
* @return void
*/
public function testValidationDefault()
{
$this->markTestIncomplete('Not implemented yet.');
}
/**
* Test buildRules method
*
* @return void
*/
public function testBuildRules()
{
$this->markTestIncomplete('Not implemented yet.');
}
}
| {'content_hash': 'fe21b81d936a063c79b273b6a17d6106', 'timestamp': '', 'source': 'github', 'line_count': 84, 'max_line_length': 91, 'avg_line_length': 17.55952380952381, 'alnum_prop': 0.5369491525423729, 'repo_name': 'Ashrafdev/rooms_crud_assessment', 'id': 'b0bb900bd993304fbb459f8a95f0aaf23ce4b651', 'size': '1475', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'tests/TestCase/Model/Table/RoomsTableTest.php', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'ApacheConf', 'bytes': '433'}, {'name': 'Batchfile', 'bytes': '834'}, {'name': 'CSS', 'bytes': '12827'}, {'name': 'JavaScript', 'bytes': '13739'}, {'name': 'PHP', 'bytes': '110910'}, {'name': 'Shell', 'bytes': '1457'}]} |
<?php
namespace Analogue\ORM\System\Proxies;
use Analogue\ORM\Mappable;
interface ProxyInterface
{
/**
* Convert a proxy into the underlying related Object
*
* @return Mappable|\Analogue\ORM\EntityCollection
*/
public function load();
/**
* Return true if the underlying relation has been lazy loaded
*
* @return boolean
*/
public function isLoaded();
}
| {'content_hash': '77c124857c297fe416db7da21c294e38', 'timestamp': '', 'source': 'github', 'line_count': 21, 'max_line_length': 66, 'avg_line_length': 19.666666666666668, 'alnum_prop': 0.648910411622276, 'repo_name': 'smallhadroncollider/analogue', 'id': 'feb89d6ceec6ab81e0f02dc7788d64861bc6e2c7', 'size': '413', 'binary': False, 'copies': '1', 'ref': 'refs/heads/5.1', 'path': 'src/System/Proxies/ProxyInterface.php', 'mode': '33261', 'license': 'mit', 'language': [{'name': 'PHP', 'bytes': '344712'}]} |
<?xml version="1.0" encoding="utf-8"?>
<PreferenceScreen
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto">
<Preference
android:key="pref_disable_battery_optimization"
android:title="@string/pref_disable_battery_optimization"
android:summary="@string/pref_disable_battery_optimization_summary"
android:icon="@drawable/ic_exclamation"
android:shouldDisableView="true"
app:isPreferenceVisible="false" />
<com.apps.adrcotfas.goodtime.settings.DayOfWeekPreference
app:key="pref_reminder_days"
app:layout="@layout/preference_days_of_week"
app:allowDividerAbove="false"
app:iconSpaceReserved="false"/>
<Preference
app:key="pref_reminder_time"
app:title="@string/reminder_time"
android:dependency="pref_reminder_days"
app:layout="@layout/preference_compact"
app:iconSpaceReserved="false"/>
<PreferenceCategory
android:title="@string/pref_header_general"
app:iconSpaceReserved="false">
<Preference
android:key="pref_timer_duration"
app:fragment="com.apps.adrcotfas.goodtime.settings.DurationsSettingsFragment"
android:title="@string/pref_timer_duration"
android:summary="@string/timer_durations"
android:icon="@drawable/ic_status_goodtime"/>
<Preference
app:key="pref_work_day_start"
app:title="@string/pref_work_day_start"
app:layout="@layout/preference_compact"
app:iconSpaceReserved="false"/>
<ListPreference
android:dialogTitle="@string/pref_timer_style"
android:key="pref_timer_style"
android:entries="@array/pref_timer_style"
android:defaultValue="@string/pref_timer_style_default_value"
android:entryValues="@array/pref_timer_style_values"
android:persistent="true"
android:summary="%s"
android:title="@string/pref_timer_style"
app:iconSpaceReserved="false"/>
<CheckBoxPreference
android:defaultValue="false"
android:key="pref_fullscreen"
android:title="@string/pref_fullscreen"
app:iconSpaceReserved="false"/>
<CheckBoxPreference
android:defaultValue="true"
android:key="pref_keep_screen_on"
android:title="@string/pref_keep_screen_on"
app:iconSpaceReserved="false"/>
<CheckBoxPreference
android:defaultValue="false"
android:dependency="pref_keep_screen_on"
android:key="pref_screen_saver"
android:title="@string/pref_screen_saver"
android:summary="@string/pref_screen_saver_summary"
app:iconSpaceReserved="false"/>
<SwitchPreferenceCompat
android:defaultValue="true"
android:persistent="true"
android:key="pref_amoled"
android:title="@string/pref_amoled"
app:iconSpaceReserved="false"/>
<CheckBoxPreference
android:defaultValue="true"
android:key="pref_sessions_counter"
android:persistent="true"
android:title="@string/pref_session_counter"
android:summary="@string/pref_session_counter_summary"
app:iconSpaceReserved="false"/>
<CheckBoxPreference
android:defaultValue="false"
android:key="pref_show_label"
android:persistent="true"
android:title="@string/pref_show_label"
android:summary="@string/pref_show_label_summary"
app:iconSpaceReserved="false"/>
</PreferenceCategory>
<PreferenceCategory
android:title="@string/pref_header_notifications"
app:iconSpaceReserved="false">
<SwitchPreferenceCompat
android:defaultValue="true"
android:key="pref_enable_ringtone"
android:title="@string/pref_enable_ringtone"
android:icon="@drawable/ic_notifications"/>
<SwitchPreferenceCompat
android:defaultValue="false"
android:key="pref_priority_alarm"
android:title="@string/pref_priority_alarm"
android:summary="@string/pref_priority_alarm_summary"
android:dependency="pref_enable_ringtone"
app:iconSpaceReserved="false"/>
<Preference
android:key="pref_notification_sound_work"
android:summary="@string/pref_ringtone_summary"
android:title="@string/pref_ringtone"
android:dependency="pref_enable_ringtone"
app:layout="@layout/preference_default"
app:iconSpaceReserved="false"/>
<Preference
android:key="pref_notification_sound_break"
android:summary="@string/pref_ringtone_summary"
android:title="@string/pref_ringtone_break"
android:dependency="pref_enable_ringtone"
app:layout="@layout/preference_default"
app:iconSpaceReserved="false"/>
<ListPreference
android:key="pref_vibration_type"
android:defaultValue="@string/pref_vibration_values_strong"
android:entryValues="@array/pref_vibration_values"
android:entries="@array/pref_vibration_types"
android:title="@string/pref_vibrate"
android:summary="%s"
android:icon="@drawable/ic_vibration"/>
<SwitchPreferenceCompat
android:defaultValue="false"
android:key="pref_flashing_notification"
android:title="@string/pref_flashing_notification"
android:summary="@string/pref_flashing_notification_summary"
android:icon="@drawable/ic_flash"/>
<SwitchPreferenceCompat
android:defaultValue="false"
android:key="pref_one_minute_left_notification"
android:title="@string/pref_one_minute_left_notification"
android:summary="@string/pref_one_minute_left_notification_summary"
app:iconSpaceReserved="false">
</SwitchPreferenceCompat>
<CheckBoxPreference
android:defaultValue="false"
android:key="pref_auto_start_break"
android:title="@string/pref_auto_start_break"
android:summary="@string/pref_auto_start_break_summary"
app:iconSpaceReserved="false"/>
<CheckBoxPreference
android:defaultValue="false"
android:key="pref_auto_start_work"
android:title="@string/pref_auto_start_work"
android:summary="@string/pref_auto_start_work_summary"
app:iconSpaceReserved="false"/>
<CheckBoxPreference
android:defaultValue="false"
android:key="pref_ringtone_insistent"
android:title="@string/pref_ringtone_insistent"
android:summary="@string/pref_ringtone_insistent_summary"
app:iconSpaceReserved="false"/>
</PreferenceCategory>
<PreferenceCategory
android:title="@string/pref_header_during_work_sessions"
app:iconSpaceReserved="false">
<CheckBoxPreference
android:defaultValue="false"
android:key="pref_disable_sound_and_vibration"
android:title="@string/pref_disable_sound_and_vibration"
app:iconSpaceReserved="false"/>
<CheckBoxPreference
android:defaultValue="false"
android:key="pref_dnd"
android:title="@string/pref_dnd"
app:iconSpaceReserved="false"/>
<CheckBoxPreference
android:defaultValue="false"
android:key="pref_disable_wifi"
android:title="@string/pref_disable_wifi"
app:iconSpaceReserved="false"/>
</PreferenceCategory>
</PreferenceScreen> | {'content_hash': '2fcf81fa357e196400adedd832102b03', 'timestamp': '', 'source': 'github', 'line_count': 203, 'max_line_length': 89, 'avg_line_length': 39.10344827586207, 'alnum_prop': 0.6242126480221718, 'repo_name': 'adrcotfas/Goodtime', 'id': '4728c4ffabe6278be2a94ad6bc091cab29a954e2', 'size': '7938', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'app/src/main/res/xml/settings.xml', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Java', 'bytes': '369452'}]} |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>equations: Not compatible 👼</title>
<link rel="shortcut icon" type="image/png" href="../../../../../favicon.png" />
<link href="../../../../../bootstrap.min.css" rel="stylesheet">
<link href="../../../../../bootstrap-custom.css" rel="stylesheet">
<link href="//maxcdn.bootstrapcdn.com/font-awesome/4.2.0/css/font-awesome.min.css" rel="stylesheet">
<script src="../../../../../moment.min.js"></script>
<!-- HTML5 Shim and Respond.js IE8 support of HTML5 elements and media queries -->
<!-- WARNING: Respond.js doesn't work if you view the page via file:// -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/html5shiv/3.7.2/html5shiv.min.js"></script>
<script src="https://oss.maxcdn.com/respond/1.4.2/respond.min.js"></script>
<![endif]-->
</head>
<body>
<div class="container">
<div class="navbar navbar-default" role="navigation">
<div class="container-fluid">
<div class="navbar-header">
<a class="navbar-brand" href="../../../../.."><i class="fa fa-lg fa-flag-checkered"></i> Coq bench</a>
</div>
<div id="navbar" class="collapse navbar-collapse">
<ul class="nav navbar-nav">
<li><a href="../..">clean / released</a></li>
<li class="active"><a href="">8.13.2 / equations - 1.2.3+8.12</a></li>
</ul>
</div>
</div>
</div>
<div class="article">
<div class="row">
<div class="col-md-12">
<a href="../..">« Up</a>
<h1>
equations
<small>
1.2.3+8.12
<span class="label label-info">Not compatible 👼</span>
</small>
</h1>
<p>📅 <em><script>document.write(moment("2022-10-06 11:12:50 +0000", "YYYY-MM-DD HH:mm:ss Z").fromNow());</script> (2022-10-06 11:12:50 UTC)</em><p>
<h2>Context</h2>
<pre># Packages matching: installed
# Name # Installed # Synopsis
base-bigarray base
base-threads base
base-unix base
conf-findutils 1 Virtual package relying on findutils
conf-gmp 4 Virtual package relying on a GMP lib system installation
coq 8.13.2 Formal proof management system
num 1.4 The legacy Num library for arbitrary-precision integer and rational arithmetic
ocaml 4.06.1 The OCaml compiler (virtual package)
ocaml-base-compiler 4.06.1 Official 4.06.1 release
ocaml-config 1 OCaml Switch Configuration
ocamlfind 1.9.5 A library manager for OCaml
zarith 1.12 Implements arithmetic and logical operations over arbitrary-precision integers
# opam file:
opam-version: "2.0"
authors: [ "Matthieu Sozeau <[email protected]>" "Cyprien Mangin <[email protected]>" ]
dev-repo: "git+https://github.com/mattam82/Coq-Equations.git#8.12"
maintainer: "[email protected]"
homepage: "https://mattam82.github.io/Coq-Equations"
bug-reports: "https://github.com/mattam82/Coq-Equations/issues"
license: "LGPL-2.1-only"
synopsis: "A function definition package for Coq"
description: """
Equations is a function definition plugin for Coq, that allows the
definition of functions by dependent pattern-matching and well-founded,
mutual or nested structural recursion and compiles them into core
terms. It automatically derives the clauses equations, the graph of the
function and its associated elimination principle.
"""
tags: [
"keyword:dependent pattern-matching"
"keyword:functional elimination"
"category:Miscellaneous/Coq Extensions"
"logpath:Equations"
]
build: [
["./configure.sh"]
[make "-j%{jobs}%"]
]
install: [
[make "install"]
]
run-test: [
[make "test-suite"]
]
depends: [
"coq" {>= "8.12.0" & < "8.13~"}
]
url {
src:
"https://github.com/mattam82/Coq-Equations/archive/v1.2.3-8.12.tar.gz"
checksum: "sha512=c19617ef127a56916d67b2c347f21cd267b250a23988424bb1486336e784a5df7a6c85ee355eb99e148431f8d7b3534993f3fdba3aaa4769d74f8c3548fb2efa"
}
</pre>
<h2>Lint</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Dry install 🏜️</h2>
<p>Dry install with the current Coq version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam install -y --show-action coq-equations.1.2.3+8.12 coq.8.13.2</code></dd>
<dt>Return code</dt>
<dd>5120</dd>
<dt>Output</dt>
<dd><pre>[NOTE] Package coq is already installed (current version is 8.13.2).
The following dependencies couldn't be met:
- coq-equations -> coq < 8.13~ -> ocaml < 4.06.0
base of this switch (use `--unlock-base' to force)
Your request can't be satisfied:
- No available version of coq satisfies the constraints
No solution found, exiting
</pre></dd>
</dl>
<p>Dry install without Coq/switch base, to test if the problem was incompatibility with the current Coq/OCaml version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam remove -y coq; opam install -y --show-action --unlock-base coq-equations.1.2.3+8.12</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Install dependencies</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>0 s</dd>
</dl>
<h2>Install 🚀</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>0 s</dd>
</dl>
<h2>Installation size</h2>
<p>No files were installed.</p>
<h2>Uninstall 🧹</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Missing removes</dt>
<dd>
none
</dd>
<dt>Wrong removes</dt>
<dd>
none
</dd>
</dl>
</div>
</div>
</div>
<hr/>
<div class="footer">
<p class="text-center">
Sources are on <a href="https://github.com/coq-bench">GitHub</a> © Guillaume Claret 🐣
</p>
</div>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
<script src="../../../../../bootstrap.min.js"></script>
</body>
</html>
| {'content_hash': '5d5f809c6ce7cd50db50095ff0c7d2a2', 'timestamp': '', 'source': 'github', 'line_count': 181, 'max_line_length': 159, 'avg_line_length': 41.149171270718234, 'alnum_prop': 0.5617615467239527, 'repo_name': 'coq-bench/coq-bench.github.io', 'id': '3f2d530f11b6a1ee8cdee11d728488f7e2d9593c', 'size': '7473', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'clean/Linux-x86_64-4.06.1-2.0.5/released/8.13.2/equations/1.2.3+8.12.html', 'mode': '33188', 'license': 'mit', 'language': []} |
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context="huyifei.mymvp.test.HashMapActivity">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="5dp"
android:orientation="horizontal">
<TextView
android:textSize="25sp"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:gravity="center"
android:padding="5dp"
android:text="key: "/>
<EditText
android:id="@+id/key"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_margin="5dp"
android:layout_weight="3"
android:padding="5dp"/>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="5dp"
android:orientation="horizontal">
<TextView
android:textSize="25sp"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:gravity="center"
android:padding="5dp"
android:text="value: "/>
<EditText
android:id="@+id/value"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_margin="5dp"
android:layout_weight="3"
android:padding="5dp"/>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="5dp"
android:orientation="horizontal">
<Button
android:id="@+id/put"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_margin="5dp"
android:layout_weight="1"
android:text="put"/>
<Button
android:id="@+id/get"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_margin="5dp"
android:layout_weight="1"
android:text="get"/>
<Button
android:id="@+id/remove"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_margin="5dp"
android:layout_weight="1"
android:text="remove"/>
</LinearLayout>
<TextView
android:id="@+id/result"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="5dp"/>
</LinearLayout>
| {'content_hash': '08f130df325fe75e3e53716bd352f528', 'timestamp': '', 'source': 'github', 'line_count': 101, 'max_line_length': 62, 'avg_line_length': 29.693069306930692, 'alnum_prop': 0.5721907302434145, 'repo_name': 'REBOOTERS/My-MVP', 'id': '32958a18304064f89b5ccae66ae1bc73d1cd0162', 'size': '2999', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'app/src/main/res/layout/activity_hash_map.xml', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Java', 'bytes': '346749'}, {'name': 'Kotlin', 'bytes': '1979'}]} |
@{
# Script module or binary module file associated with this manifest.
RootModule = 'SBAuthorizationRule.psm1'
# Version number of this module.
ModuleVersion = '0.10.1.0'
# Supported PSEditions
# CompatiblePSEditions = @()
# ID used to uniquely identify this module
GUID = '286b166a-9bff-4ed0-859a-b22ff26a4733'
# Author of this module
Author = 'Ryan Spletzer'
# Company or vendor of this module
CompanyName = ''
# Copyright statement for this module
Copyright = '(c) 2017 Ryan Spletzer. All rights reserved.'
# Description of the functionality provided by this module
# Description = ''
# Minimum version of the Windows PowerShell engine required by this module
PowerShellVersion = '5.0'
# Name of the Windows PowerShell host required by this module
# PowerShellHostName = ''
# Minimum version of the Windows PowerShell host required by this module
# PowerShellHostVersion = ''
# Minimum version of Microsoft .NET Framework required by this module. This prerequisite is valid for the PowerShell Desktop edition only.
# DotNetFrameworkVersion = ''
# Minimum version of the common language runtime (CLR) required by this module. This prerequisite is valid for the PowerShell Desktop edition only.
# CLRVersion = ''
# Processor architecture (None, X86, Amd64) required by this module
# ProcessorArchitecture = ''
# Modules that must be imported into the global environment prior to importing this module
# RequiredModules = @()
# Assemblies that must be loaded prior to importing this module
# RequiredAssemblies = @()
# Script files (.ps1) that are run in the caller's environment prior to importing this module.
# ScriptsToProcess = @()
# Type files (.ps1xml) to be loaded when importing this module
# TypesToProcess = @()
# Format files (.ps1xml) to be loaded when importing this module
# FormatsToProcess = @()
# Modules to import as nested modules of the module specified in RootModule/ModuleToProcess
NestedModules = @( '..\..\Modules\SB.Util\SB.Util.psd1' )
# Functions to export from this module, for best performance, do not use wildcards and do not delete the entry, use an empty array if there are no functions to export.
# FunctionsToExport = @()
# Cmdlets to export from this module, for best performance, do not use wildcards and do not delete the entry, use an empty array if there are no cmdlets to export.
# CmdletsToExport = @()
# Variables to export from this module
# VariablesToExport = '*'
# Aliases to export from this module, for best performance, do not use wildcards and do not delete the entry, use an empty array if there are no aliases to export.
# AliasesToExport = @()
# DSC resources to export from this module
DscResourcesToExport = 'SBAuthorizationRule'
# List of all modules packaged with this module
# ModuleList = @()
# List of all files packaged with this module
# FileList = @()
# HelpInfo URI of this module
# HelpInfoURI = ''
# Default prefix for commands exported from this module. Override the default prefix using Import-Module -Prefix.
# DefaultCommandPrefix = ''
}
| {'content_hash': '16df810e13882c4c6225e3e467cb0adb', 'timestamp': '', 'source': 'github', 'line_count': 90, 'max_line_length': 167, 'avg_line_length': 33.77777777777778, 'alnum_prop': 0.7578947368421053, 'repo_name': 'ryanspletzer/ServiceBusForWindowsServerDsc', 'id': 'ab3ef3521193e63bc1ccca8b175bf0f29cfdce38', 'size': '3040', 'binary': False, 'copies': '1', 'ref': 'refs/heads/dev', 'path': 'DSCClassResources/SBAuthorizationRule/SBAuthorizationRule.psd1', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'PowerShell', 'bytes': '289128'}]} |
package org.jboss.pnc.executor;
import org.jboss.pnc.common.Configuration;
import org.jboss.pnc.common.json.ConfigurationParseException;
import org.jboss.pnc.common.json.moduleconfig.SystemConfig;
import org.jboss.pnc.common.json.moduleprovider.PncConfigProvider;
import org.jboss.pnc.executor.exceptions.BuildProcessException;
import org.jboss.pnc.executor.servicefactories.BuildDriverFactory;
import org.jboss.pnc.executor.servicefactories.EnvironmentDriverFactory;
import org.jboss.pnc.executor.servicefactories.RepositoryManagerFactory;
import org.jboss.pnc.model.RepositoryType;
import org.jboss.pnc.spi.BuildExecutionStatus;
import org.jboss.pnc.spi.builddriver.BuildDriver;
import org.jboss.pnc.spi.builddriver.BuildDriverResult;
import org.jboss.pnc.spi.builddriver.BuildDriverStatus;
import org.jboss.pnc.spi.builddriver.CompletedBuild;
import org.jboss.pnc.spi.builddriver.RunningBuild;
import org.jboss.pnc.spi.environment.DestroyableEnvironment;
import org.jboss.pnc.spi.environment.EnvironmentDriver;
import org.jboss.pnc.spi.environment.RunningEnvironment;
import org.jboss.pnc.spi.environment.StartedEnvironment;
import org.jboss.pnc.spi.environment.exception.EnvironmentDriverException;
import org.jboss.pnc.spi.events.BuildExecutionStatusChangedEvent;
import org.jboss.pnc.spi.executor.BuildExecutionConfiguration;
import org.jboss.pnc.spi.executor.BuildExecutionSession;
import org.jboss.pnc.spi.executor.BuildExecutor;
import org.jboss.pnc.spi.executor.exceptions.ExecutorException;
import org.jboss.pnc.spi.repositorymanager.BuildExecution;
import org.jboss.pnc.spi.repositorymanager.RepositoryManager;
import org.jboss.pnc.spi.repositorymanager.RepositoryManagerResult;
import org.jboss.pnc.spi.repositorymanager.model.RepositorySession;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import javax.annotation.PreDestroy;
import javax.enterprise.context.ApplicationScoped;
import javax.inject.Inject;
import java.net.URI;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
import java.util.Optional;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.function.Consumer;
/**
* @author <a href="mailto:[email protected]">Matej Lazar</a>
*/
@ApplicationScoped
public class DefaultBuildExecutor implements BuildExecutor {
private final Logger log = LoggerFactory.getLogger(DefaultBuildExecutor.class);
private ExecutorService executor;
private RepositoryManagerFactory repositoryManagerFactory;
private BuildDriverFactory buildDriverFactory;
private EnvironmentDriverFactory environmentDriverFactory;
private final Map<Integer, BuildExecutionSession> runningExecutions = new HashMap<>();
@Deprecated
public DefaultBuildExecutor() {}; //CDI workaround for constructor injection
@Inject
public DefaultBuildExecutor(
RepositoryManagerFactory repositoryManagerFactory,
BuildDriverFactory buildDriverFactory,
EnvironmentDriverFactory environmentDriverFactory,
Configuration configuration) {
this.repositoryManagerFactory = repositoryManagerFactory;
this.buildDriverFactory = buildDriverFactory;
this.environmentDriverFactory = environmentDriverFactory;
int executorThreadPoolSize = 12;
try {
String executorThreadPoolSizeStr = configuration.getModuleConfig(new PncConfigProvider<>(SystemConfig.class)).getExecutorThreadPoolSize();
if (executorThreadPoolSizeStr != null) {
executorThreadPoolSize = Integer.parseInt(executorThreadPoolSizeStr);
}
} catch (ConfigurationParseException e) {
log.warn("Unable parse config. Using defaults.");
}
executor = Executors.newFixedThreadPool(executorThreadPoolSize);
}
@Override
public BuildExecutionSession startBuilding(
BuildExecutionConfiguration buildExecutionConfiguration,
Consumer<BuildExecutionStatusChangedEvent> onBuildExecutionStatusChangedEvent) throws ExecutorException {
BuildExecutionSession buildExecutionSession = new DefaultBuildExecutionSession(buildExecutionConfiguration, onBuildExecutionStatusChangedEvent);
buildExecutionSession.setStatus(BuildExecutionStatus.NEW);
//TODO update logging: log.info("Staring build execution task: {}; Build Configuration id: {}.", buildExecutionConfiguration.getId(), buildExecutionConfiguration.etBuildConfiguration().getId());
runningExecutions.put(buildExecutionConfiguration.getId(), buildExecutionSession);
CompletableFuture.supplyAsync(() -> configureRepository(buildExecutionSession), executor)
.thenApplyAsync(repositoryConfiguration -> setUpEnvironment(buildExecutionSession, repositoryConfiguration), executor)
.thenComposeAsync(startedEnvironment -> waitForEnvironmentInitialization(buildExecutionSession, startedEnvironment), executor)
.thenApplyAsync(nul -> buildSetUp(buildExecutionSession), executor)
.thenComposeAsync(runningBuild -> waitBuildToComplete(buildExecutionSession, runningBuild), executor)
.thenApplyAsync(completedBuild -> retrieveBuildDriverResults(buildExecutionSession, completedBuild), executor)
.thenApplyAsync(nul -> retrieveRepositoryManagerResults(buildExecutionSession), executor)
.thenApplyAsync(nul -> destroyEnvironment(buildExecutionSession), executor)
.handleAsync((nul, e) -> completeExecution(buildExecutionSession, e), executor);
//TODO re-connect running instances in case of crash
return buildExecutionSession;
}
@Override
public BuildExecutionSession getRunningExecution(int buildExecutionTaskId) {
return runningExecutions.get(buildExecutionTaskId);
}
private RepositorySession configureRepository(BuildExecutionSession buildExecutionSession) {
buildExecutionSession.setStatus(BuildExecutionStatus.REPO_SETTING_UP);
try {
RepositoryManager repositoryManager = repositoryManagerFactory.getRepositoryManager(RepositoryType.MAVEN);
BuildExecution buildExecution = buildExecutionSession.getBuildExecutionConfiguration();
return repositoryManager.createBuildRepository(buildExecution);
} catch (Throwable e) {
throw new BuildProcessException(e);
}
}
private StartedEnvironment setUpEnvironment(BuildExecutionSession buildExecutionSession, RepositorySession repositorySession) {
buildExecutionSession.setStatus(BuildExecutionStatus.BUILD_ENV_SETTING_UP);
BuildExecutionConfiguration buildExecutionConfiguration = buildExecutionSession.getBuildExecutionConfiguration();
try {
EnvironmentDriver envDriver = environmentDriverFactory.getDriver(buildExecutionConfiguration.getBuildType());
StartedEnvironment startedEnv = envDriver.buildEnvironment(
buildExecutionConfiguration.getBuildType(),
repositorySession);
return startedEnv;
} catch (Throwable e) {
throw new BuildProcessException(e);
}
}
private CompletableFuture<Void> waitForEnvironmentInitialization(BuildExecutionSession buildExecutionSession, StartedEnvironment startedEnvironment) {
CompletableFuture<Void> waitToCompleteFuture = new CompletableFuture<>();
try {
Consumer<RunningEnvironment> onComplete = (runningEnvironment) -> {
buildExecutionSession.setRunningEnvironment(runningEnvironment);
buildExecutionSession.setStatus(BuildExecutionStatus.BUILD_ENV_SETUP_COMPLETE_SUCCESS);
waitToCompleteFuture.complete(null);
};
Consumer<Exception> onError = (e) -> {
buildExecutionSession.setStatus(BuildExecutionStatus.BUILD_ENV_SETUP_COMPLETE_WITH_ERROR);
waitToCompleteFuture.completeExceptionally(new BuildProcessException(e, startedEnvironment));
};
buildExecutionSession.setStatus(BuildExecutionStatus.BUILD_ENV_WAITING);
startedEnvironment.monitorInitialization(onComplete, onError);
} catch (Throwable e) {
waitToCompleteFuture.completeExceptionally(new BuildProcessException(e, startedEnvironment));
}
return waitToCompleteFuture;
}
private RunningBuild buildSetUp(BuildExecutionSession buildExecutionSession) {
buildExecutionSession.setStatus(BuildExecutionStatus.BUILD_SETTING_UP);
RunningEnvironment runningEnvironment = buildExecutionSession.getRunningEnvironment();
try {
String liveLogWebSocketUrl = runningEnvironment.getBuildAgentUrl();
log.debug("Setting live log websocket url: {}", liveLogWebSocketUrl);
buildExecutionSession.setLiveLogsUri(Optional.of(new URI(liveLogWebSocketUrl)));
buildExecutionSession.setStartTime(new Date());
BuildDriver buildDriver = buildDriverFactory.getBuildDriver(buildExecutionSession.getBuildExecutionConfiguration().getBuildType());
return buildDriver.startProjectBuild(buildExecutionSession, runningEnvironment);
} catch (Throwable e) {
throw new BuildProcessException(e, runningEnvironment);
}
}
private CompletableFuture<CompletedBuild> waitBuildToComplete(BuildExecutionSession buildExecutionSession, RunningBuild runningBuild) {
CompletableFuture<CompletedBuild> waitToCompleteFuture = new CompletableFuture<>();
try {
Consumer<CompletedBuild> onComplete = (completedBuild) -> {
waitToCompleteFuture.complete(completedBuild);
};
Consumer<Throwable> onError = (e) -> {
waitToCompleteFuture.completeExceptionally(new BuildProcessException(e, runningBuild.getRunningEnvironment()));
};
buildExecutionSession.setStatus(BuildExecutionStatus.BUILD_WAITING);
runningBuild.monitor(onComplete, onError);
} catch (Throwable exception) {
waitToCompleteFuture.completeExceptionally(
new BuildProcessException(exception, runningBuild.getRunningEnvironment()));
}
return waitToCompleteFuture;
}
private Void retrieveBuildDriverResults(BuildExecutionSession buildExecutionSession, CompletedBuild completedBuild) {
buildExecutionSession.setStatus(BuildExecutionStatus.COLLECTING_RESULTS_FROM_BUILD_DRIVER);
try {
BuildDriverResult buildResult = completedBuild.getBuildResult();
BuildDriverStatus buildDriverStatus = buildResult.getBuildDriverStatus();
buildExecutionSession.setBuildDriverResult(buildResult);
if (buildDriverStatus.completedSuccessfully()) {
buildExecutionSession.setStatus(BuildExecutionStatus.BUILD_COMPLETED_SUCCESS);
} else {
buildExecutionSession.setStatus(BuildExecutionStatus.BUILD_COMPLETED_WITH_ERROR);
}
return null;
} catch (Throwable e) {
throw new BuildProcessException(e, completedBuild.getRunningEnvironment());
}
}
private Void retrieveRepositoryManagerResults(BuildExecutionSession buildExecutionSession) {
try {
buildExecutionSession.setStatus(BuildExecutionStatus.COLLECTING_RESULTS_FROM_REPOSITORY_NAMAGER);
RunningEnvironment runningEnvironment = buildExecutionSession.getRunningEnvironment();
buildExecutionSession.setRunningEnvironment(runningEnvironment);
RepositorySession repositorySession = runningEnvironment.getRepositorySession();
RepositoryManagerResult repositoryManagerResult = repositorySession.extractBuildArtifacts();
buildExecutionSession.setRepositoryManagerResult(repositoryManagerResult);
} catch (Throwable e) {
throw new BuildProcessException(e, buildExecutionSession.getRunningEnvironment());
}
return null;
}
private Void destroyEnvironment(BuildExecutionSession buildExecutionSession) {
try {
buildExecutionSession.setStatus(BuildExecutionStatus.BUILD_ENV_DESTROYING);
buildExecutionSession.getRunningEnvironment().destroyEnvironment();
buildExecutionSession.setStatus(BuildExecutionStatus.BUILD_ENV_DESTROYED);
} catch (Throwable e) {
throw new BuildProcessException(e);
}
return null;
}
private Void completeExecution(BuildExecutionSession buildExecutionSession, Throwable e) {
buildExecutionSession.setStatus(BuildExecutionStatus.FINALIZING_EXECUTION);
if (buildExecutionSession.getStartTime() == null) {
buildExecutionSession.setException(new ExecutorException("Missing start time."));
}
if (e != null) {
stopRunningEnvironment(e);
}
if (e != null) {
buildExecutionSession.setException(new ExecutorException(e));
}
if (buildExecutionSession.getEndTime() != null) {
buildExecutionSession.setException(new ExecutorException("End time already set."));
} else {
buildExecutionSession.setEndTime(new Date());
}
//check if any of previous statuses indicated "failed" state
if (buildExecutionSession.hasFailed()) { //TODO differentiate build and system error
buildExecutionSession.setStatus(BuildExecutionStatus.DONE_WITH_ERRORS);
} else {
buildExecutionSession.setStatus(BuildExecutionStatus.DONE);
}
log.debug("Removing buildExecutionTask [" + buildExecutionSession.getId() + "] form list of running tasks.");
runningExecutions.remove(buildExecutionSession.getId());
return null;
}
/**
* Tries to stop running environment if the exception contains information about running environment
*
* @param ex Exception in build process (To stop the environment it has to be instance of BuildProcessException)
*/
private void stopRunningEnvironment(Throwable ex) {
DestroyableEnvironment destroyableEnvironment = null;
if(ex instanceof BuildProcessException) {
BuildProcessException bpEx = (BuildProcessException) ex;
destroyableEnvironment = bpEx.getDestroyableEnvironment();
} else if(ex.getCause() instanceof BuildProcessException) {
BuildProcessException bpEx = (BuildProcessException) ex.getCause();
destroyableEnvironment = bpEx.getDestroyableEnvironment();
} else {
//It shouldn't never happen - Throwable should be caught in all steps of build chain
//and BuildProcessException should be thrown instead of that
log.warn("Possible leak of a running environment! Build process ended with exception, "
+ "but the exception didn't contain information about running environment.", ex);
}
try {
if (destroyableEnvironment != null)
destroyableEnvironment.destroyEnvironment();
} catch (EnvironmentDriverException envE) {
log.warn("Running environment" + destroyableEnvironment + " couldn't be destroyed!", envE);
}
}
@Override
@PreDestroy
public void shutdown() {
executor.shutdown();
}
}
| {'content_hash': '9d8cef4a54935e9a7b11d423fc787cd0', 'timestamp': '', 'source': 'github', 'line_count': 315, 'max_line_length': 202, 'avg_line_length': 49.34603174603175, 'alnum_prop': 0.7321796191456511, 'repo_name': 'pgier/pnc', 'id': '72b298e7dfad469a5c27f62310d6a82e3fe43049', 'size': '16247', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'build-executor/src/main/java/org/jboss/pnc/executor/DefaultBuildExecutor.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'CSS', 'bytes': '102097'}, {'name': 'Groovy', 'bytes': '884'}, {'name': 'HTML', 'bytes': '204866'}, {'name': 'Java', 'bytes': '1948630'}, {'name': 'JavaScript', 'bytes': '2724526'}, {'name': 'Shell', 'bytes': '7028'}]} |
package mil.nga.giat.mage.sdk.event;
public interface IUserDispatcher {
/**
* Adds a listener
*
* @param listener
* @return
* @throws Exception
*/
boolean addListener(final IUserEventListener listener) throws Exception;
/**
* Removes the listener
*
* @param listener
* @return
* @throws Exception
*/
boolean removeListener(final IUserEventListener listener) throws Exception;
}
| {'content_hash': '3a6200d38c7839b97393897632566076', 'timestamp': '', 'source': 'github', 'line_count': 22, 'max_line_length': 79, 'avg_line_length': 18.954545454545453, 'alnum_prop': 0.6930455635491607, 'repo_name': 'ngageoint/mage-android', 'id': 'abecbc68d37d70d5aed5c477f9c7cb874d3f6cba', 'size': '417', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'mage/src/main/java/mil/nga/giat/mage/sdk/event/IUserDispatcher.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Java', 'bytes': '654440'}, {'name': 'Kotlin', 'bytes': '906967'}]} |
<menu xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
tools:context="com.hmsoft.weargoproremote.ui.TestActivity">
<item android:id="@+id/action_video_vga60" android:title="@string/action_video_vga60"
android:orderInCategory="100" android:showAsAction="never" />
<item android:id="@+id/action_video_72030" android:title="@string/action_video_72030"
android:orderInCategory="100" android:showAsAction="never" />
<item android:id="@+id/action_video_720g60" android:title="@string/action_video_72060"
android:orderInCategory="100" android:showAsAction="never" />
<item android:id="@+id/action_video_96030" android:title="@string/action_video_96030"
android:orderInCategory="100" android:showAsAction="never" />
<item android:id="@+id/action_video_108030" android:title="@string/action_video_108030"
android:orderInCategory="100" android:showAsAction="never" />
</menu> | {'content_hash': 'd45573106dd72af6bd18e93246c54593', 'timestamp': '', 'source': 'github', 'line_count': 14, 'max_line_length': 91, 'avg_line_length': 70.71428571428571, 'alnum_prop': 0.7202020202020202, 'repo_name': 'hmrs-cr/android-wear-gopro-remote', 'id': '1ece3a71209dcc01a6822e796c70e5f02a31e118', 'size': '990', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'mobile/src/main/res/menu/menu_video_modes.xml', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Java', 'bytes': '185088'}]} |
/*[clinic input]
preserve
[clinic start generated code]*/
PyDoc_STRVAR(_io_open__doc__,
"open($module, /, file, mode=\'r\', buffering=-1, encoding=None,\n"
" errors=None, newline=None, closefd=True, opener=None)\n"
"--\n"
"\n"
"Open file and return a stream. Raise OSError upon failure.\n"
"\n"
"file is either a text or byte string giving the name (and the path\n"
"if the file isn\'t in the current working directory) of the file to\n"
"be opened or an integer file descriptor of the file to be\n"
"wrapped. (If a file descriptor is given, it is closed when the\n"
"returned I/O object is closed, unless closefd is set to False.)\n"
"\n"
"mode is an optional string that specifies the mode in which the file\n"
"is opened. It defaults to \'r\' which means open for reading in text\n"
"mode. Other common values are \'w\' for writing (truncating the file if\n"
"it already exists), \'x\' for creating and writing to a new file, and\n"
"\'a\' for appending (which on some Unix systems, means that all writes\n"
"append to the end of the file regardless of the current seek position).\n"
"In text mode, if encoding is not specified the encoding used is platform\n"
"dependent: locale.getpreferredencoding(False) is called to get the\n"
"current locale encoding. (For reading and writing raw bytes use binary\n"
"mode and leave encoding unspecified.) The available modes are:\n"
"\n"
"========= ===============================================================\n"
"Character Meaning\n"
"--------- ---------------------------------------------------------------\n"
"\'r\' open for reading (default)\n"
"\'w\' open for writing, truncating the file first\n"
"\'x\' create a new file and open it for writing\n"
"\'a\' open for writing, appending to the end of the file if it exists\n"
"\'b\' binary mode\n"
"\'t\' text mode (default)\n"
"\'+\' open a disk file for updating (reading and writing)\n"
"\'U\' universal newline mode (deprecated)\n"
"========= ===============================================================\n"
"\n"
"The default mode is \'rt\' (open for reading text). For binary random\n"
"access, the mode \'w+b\' opens and truncates the file to 0 bytes, while\n"
"\'r+b\' opens the file without truncation. The \'x\' mode implies \'w\' and\n"
"raises an `FileExistsError` if the file already exists.\n"
"\n"
"Python distinguishes between files opened in binary and text modes,\n"
"even when the underlying operating system doesn\'t. Files opened in\n"
"binary mode (appending \'b\' to the mode argument) return contents as\n"
"bytes objects without any decoding. In text mode (the default, or when\n"
"\'t\' is appended to the mode argument), the contents of the file are\n"
"returned as strings, the bytes having been first decoded using a\n"
"platform-dependent encoding or using the specified encoding if given.\n"
"\n"
"\'U\' mode is deprecated and will raise an exception in future versions\n"
"of Python. It has no effect in Python 3. Use newline to control\n"
"universal newlines mode.\n"
"\n"
"buffering is an optional integer used to set the buffering policy.\n"
"Pass 0 to switch buffering off (only allowed in binary mode), 1 to select\n"
"line buffering (only usable in text mode), and an integer > 1 to indicate\n"
"the size of a fixed-size chunk buffer. When no buffering argument is\n"
"given, the default buffering policy works as follows:\n"
"\n"
"* Binary files are buffered in fixed-size chunks; the size of the buffer\n"
" is chosen using a heuristic trying to determine the underlying device\'s\n"
" \"block size\" and falling back on `io.DEFAULT_BUFFER_SIZE`.\n"
" On many systems, the buffer will typically be 4096 or 8192 bytes long.\n"
"\n"
"* \"Interactive\" text files (files for which isatty() returns True)\n"
" use line buffering. Other text files use the policy described above\n"
" for binary files.\n"
"\n"
"encoding is the name of the encoding used to decode or encode the\n"
"file. This should only be used in text mode. The default encoding is\n"
"platform dependent, but any encoding supported by Python can be\n"
"passed. See the codecs module for the list of supported encodings.\n"
"\n"
"errors is an optional string that specifies how encoding errors are to\n"
"be handled---this argument should not be used in binary mode. Pass\n"
"\'strict\' to raise a ValueError exception if there is an encoding error\n"
"(the default of None has the same effect), or pass \'ignore\' to ignore\n"
"errors. (Note that ignoring encoding errors can lead to data loss.)\n"
"See the documentation for codecs.register or run \'help(codecs.Codec)\'\n"
"for a list of the permitted encoding error strings.\n"
"\n"
"newline controls how universal newlines works (it only applies to text\n"
"mode). It can be None, \'\', \'\\n\', \'\\r\', and \'\\r\\n\'. It works as\n"
"follows:\n"
"\n"
"* On input, if newline is None, universal newlines mode is\n"
" enabled. Lines in the input can end in \'\\n\', \'\\r\', or \'\\r\\n\', and\n"
" these are translated into \'\\n\' before being returned to the\n"
" caller. If it is \'\', universal newline mode is enabled, but line\n"
" endings are returned to the caller untranslated. If it has any of\n"
" the other legal values, input lines are only terminated by the given\n"
" string, and the line ending is returned to the caller untranslated.\n"
"\n"
"* On output, if newline is None, any \'\\n\' characters written are\n"
" translated to the system default line separator, os.linesep. If\n"
" newline is \'\' or \'\\n\', no translation takes place. If newline is any\n"
" of the other legal values, any \'\\n\' characters written are translated\n"
" to the given string.\n"
"\n"
"If closefd is False, the underlying file descriptor will be kept open\n"
"when the file is closed. This does not work when a file name is given\n"
"and must be True in that case.\n"
"\n"
"A custom opener can be used by passing a callable as *opener*. The\n"
"underlying file descriptor for the file object is then obtained by\n"
"calling *opener* with (*file*, *flags*). *opener* must return an open\n"
"file descriptor (passing os.open as *opener* results in functionality\n"
"similar to passing None).\n"
"\n"
"open() returns a file object whose type depends on the mode, and\n"
"through which the standard file operations such as reading and writing\n"
"are performed. When open() is used to open a file in a text mode (\'w\',\n"
"\'r\', \'wt\', \'rt\', etc.), it returns a TextIOWrapper. When used to open\n"
"a file in a binary mode, the returned class varies: in read binary\n"
"mode, it returns a BufferedReader; in write binary and append binary\n"
"modes, it returns a BufferedWriter, and in read/write mode, it returns\n"
"a BufferedRandom.\n"
"\n"
"It is also possible to use a string or bytearray as a file for both\n"
"reading and writing. For strings StringIO can be used like a file\n"
"opened in a text mode, and for bytes a BytesIO can be used like a file\n"
"opened in a binary mode.");
#define _IO_OPEN_METHODDEF \
{"open", (PyCFunction)(void(*)(void))_io_open, METH_FASTCALL|METH_KEYWORDS, _io_open__doc__},
static PyObject *
_io_open_impl(PyObject *module, PyObject *file, const char *mode,
int buffering, const char *encoding, const char *errors,
const char *newline, int closefd, PyObject *opener);
static PyObject *
_io_open(PyObject *module, PyObject *const *args, Py_ssize_t nargs, PyObject *kwnames)
{
PyObject *return_value = NULL;
static const char * const _keywords[] = {"file", "mode", "buffering", "encoding", "errors", "newline", "closefd", "opener", NULL};
static _PyArg_Parser _parser = {NULL, _keywords, "open", 0};
PyObject *argsbuf[8];
Py_ssize_t noptargs = nargs + (kwnames ? PyTuple_GET_SIZE(kwnames) : 0) - 1;
PyObject *file;
const char *mode = "r";
int buffering = -1;
const char *encoding = NULL;
const char *errors = NULL;
const char *newline = NULL;
int closefd = 1;
PyObject *opener = Py_None;
args = _PyArg_UnpackKeywords(args, nargs, NULL, kwnames, &_parser, 1, 8, 0, argsbuf);
if (!args) {
goto exit;
}
file = args[0];
if (!noptargs) {
goto skip_optional_pos;
}
if (args[1]) {
if (!PyUnicode_Check(args[1])) {
_PyArg_BadArgument("open", "argument 'mode'", "str", args[1]);
goto exit;
}
Py_ssize_t mode_length;
mode = PyUnicode_AsUTF8AndSize(args[1], &mode_length);
if (mode == NULL) {
goto exit;
}
if (strlen(mode) != (size_t)mode_length) {
PyErr_SetString(PyExc_ValueError, "embedded null character");
goto exit;
}
if (!--noptargs) {
goto skip_optional_pos;
}
}
if (args[2]) {
if (PyFloat_Check(args[2])) {
PyErr_SetString(PyExc_TypeError,
"integer argument expected, got float" );
goto exit;
}
buffering = _PyLong_AsInt(args[2]);
if (buffering == -1 && PyErr_Occurred()) {
goto exit;
}
if (!--noptargs) {
goto skip_optional_pos;
}
}
if (args[3]) {
if (args[3] == Py_None) {
encoding = NULL;
}
else if (PyUnicode_Check(args[3])) {
Py_ssize_t encoding_length;
encoding = PyUnicode_AsUTF8AndSize(args[3], &encoding_length);
if (encoding == NULL) {
goto exit;
}
if (strlen(encoding) != (size_t)encoding_length) {
PyErr_SetString(PyExc_ValueError, "embedded null character");
goto exit;
}
}
else {
_PyArg_BadArgument("open", "argument 'encoding'", "str or None", args[3]);
goto exit;
}
if (!--noptargs) {
goto skip_optional_pos;
}
}
if (args[4]) {
if (args[4] == Py_None) {
errors = NULL;
}
else if (PyUnicode_Check(args[4])) {
Py_ssize_t errors_length;
errors = PyUnicode_AsUTF8AndSize(args[4], &errors_length);
if (errors == NULL) {
goto exit;
}
if (strlen(errors) != (size_t)errors_length) {
PyErr_SetString(PyExc_ValueError, "embedded null character");
goto exit;
}
}
else {
_PyArg_BadArgument("open", "argument 'errors'", "str or None", args[4]);
goto exit;
}
if (!--noptargs) {
goto skip_optional_pos;
}
}
if (args[5]) {
if (args[5] == Py_None) {
newline = NULL;
}
else if (PyUnicode_Check(args[5])) {
Py_ssize_t newline_length;
newline = PyUnicode_AsUTF8AndSize(args[5], &newline_length);
if (newline == NULL) {
goto exit;
}
if (strlen(newline) != (size_t)newline_length) {
PyErr_SetString(PyExc_ValueError, "embedded null character");
goto exit;
}
}
else {
_PyArg_BadArgument("open", "argument 'newline'", "str or None", args[5]);
goto exit;
}
if (!--noptargs) {
goto skip_optional_pos;
}
}
if (args[6]) {
if (PyFloat_Check(args[6])) {
PyErr_SetString(PyExc_TypeError,
"integer argument expected, got float" );
goto exit;
}
closefd = _PyLong_AsInt(args[6]);
if (closefd == -1 && PyErr_Occurred()) {
goto exit;
}
if (!--noptargs) {
goto skip_optional_pos;
}
}
opener = args[7];
skip_optional_pos:
return_value = _io_open_impl(module, file, mode, buffering, encoding, errors, newline, closefd, opener);
exit:
return return_value;
}
PyDoc_STRVAR(_io_open_code__doc__,
"open_code($module, /, path)\n"
"--\n"
"\n"
"Opens the provided file with the intent to import the contents.\n"
"\n"
"This may perform extra validation beyond open(), but is otherwise interchangeable\n"
"with calling open(path, \'rb\').");
#define _IO_OPEN_CODE_METHODDEF \
{"open_code", (PyCFunction)(void(*)(void))_io_open_code, METH_FASTCALL|METH_KEYWORDS, _io_open_code__doc__},
static PyObject *
_io_open_code_impl(PyObject *module, PyObject *path);
static PyObject *
_io_open_code(PyObject *module, PyObject *const *args, Py_ssize_t nargs, PyObject *kwnames)
{
PyObject *return_value = NULL;
static const char * const _keywords[] = {"path", NULL};
static _PyArg_Parser _parser = {NULL, _keywords, "open_code", 0};
PyObject *argsbuf[1];
PyObject *path;
args = _PyArg_UnpackKeywords(args, nargs, NULL, kwnames, &_parser, 1, 1, 0, argsbuf);
if (!args) {
goto exit;
}
if (!PyUnicode_Check(args[0])) {
_PyArg_BadArgument("open_code", "argument 'path'", "str", args[0]);
goto exit;
}
if (PyUnicode_READY(args[0]) == -1) {
goto exit;
}
path = args[0];
return_value = _io_open_code_impl(module, path);
exit:
return return_value;
}
/*[clinic end generated code: output=3df6bc6d91697545 input=a9049054013a1b77]*/
| {'content_hash': '615ed382050205b02525cb60879ccdde', 'timestamp': '', 'source': 'github', 'line_count': 326, 'max_line_length': 134, 'avg_line_length': 40.99386503067485, 'alnum_prop': 0.6135887458844658, 'repo_name': 'batermj/algorithm-challenger', 'id': '1a9651d340813f03dc84800f08758b897b0515b1', 'size': '13364', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'code-analysis/programming_anguage/python/source_codes/Python3.8.0/Python-3.8.0/Modules/_io/clinic/_iomodule.c.h', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Assembly', 'bytes': '655185'}, {'name': 'Batchfile', 'bytes': '127416'}, {'name': 'C', 'bytes': '33127630'}, {'name': 'C++', 'bytes': '1364796'}, {'name': 'CSS', 'bytes': '3163'}, {'name': 'Common Lisp', 'bytes': '48962'}, {'name': 'DIGITAL Command Language', 'bytes': '26402'}, {'name': 'DTrace', 'bytes': '2196'}, {'name': 'Go', 'bytes': '26248'}, {'name': 'HTML', 'bytes': '385719'}, {'name': 'Haskell', 'bytes': '33612'}, {'name': 'Java', 'bytes': '1084'}, {'name': 'JavaScript', 'bytes': '20754'}, {'name': 'M4', 'bytes': '403992'}, {'name': 'Makefile', 'bytes': '238185'}, {'name': 'Objective-C', 'bytes': '4934684'}, {'name': 'PHP', 'bytes': '3513'}, {'name': 'PLSQL', 'bytes': '45772'}, {'name': 'Perl', 'bytes': '649'}, {'name': 'PostScript', 'bytes': '27606'}, {'name': 'PowerShell', 'bytes': '21737'}, {'name': 'Python', 'bytes': '55270625'}, {'name': 'R', 'bytes': '29951'}, {'name': 'Rich Text Format', 'bytes': '14551'}, {'name': 'Roff', 'bytes': '292490'}, {'name': 'Ruby', 'bytes': '519'}, {'name': 'Scala', 'bytes': '846446'}, {'name': 'Shell', 'bytes': '491113'}, {'name': 'Swift', 'bytes': '881'}, {'name': 'TeX', 'bytes': '337654'}, {'name': 'VBScript', 'bytes': '140'}, {'name': 'XSLT', 'bytes': '153'}]} |
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<!-- NewPage -->
<html lang="en">
<head>
<!-- Generated by javadoc (version 1.7.0_11) on Mon Mar 17 10:51:57 PDT 2014 -->
<title>Uses of Class com.box.boxjavalibv2.BoxConfigBuilder</title>
<meta name="date" content="2014-03-17">
<link rel="stylesheet" type="text/css" href="../../../../stylesheet.css" title="Style">
</head>
<body>
<script type="text/javascript"><!--
if (location.href.indexOf('is-external=true') == -1) {
parent.document.title="Uses of Class com.box.boxjavalibv2.BoxConfigBuilder";
}
//-->
</script>
<noscript>
<div>JavaScript is disabled on your browser.</div>
</noscript>
<!-- ========= START OF TOP NAVBAR ======= -->
<div class="topNav"><a name="navbar_top">
<!-- -->
</a><a href="#skip-navbar_top" title="Skip navigation links"></a><a name="navbar_top_firstrow">
<!-- -->
</a>
<ul class="navList" title="Navigation">
<li><a href="../../../../overview-summary.html">Overview</a></li>
<li><a href="../package-summary.html">Package</a></li>
<li><a href="../../../../com/box/boxjavalibv2/BoxConfigBuilder.html" title="class in com.box.boxjavalibv2">Class</a></li>
<li class="navBarCell1Rev">Use</li>
<li><a href="../../../../overview-tree.html">Tree</a></li>
<li><a href="../../../../deprecated-list.html">Deprecated</a></li>
<li><a href="../../../../index-files/index-1.html">Index</a></li>
<li><a href="../../../../help-doc.html">Help</a></li>
</ul>
</div>
<div class="subNav">
<ul class="navList">
<li>Prev</li>
<li>Next</li>
</ul>
<ul class="navList">
<li><a href="../../../../index.html?com/box/boxjavalibv2/class-use/BoxConfigBuilder.html" target="_top">Frames</a></li>
<li><a href="BoxConfigBuilder.html" target="_top">No Frames</a></li>
</ul>
<ul class="navList" id="allclasses_navbar_top">
<li><a href="../../../../allclasses-noframe.html">All Classes</a></li>
</ul>
<div>
<script type="text/javascript"><!--
allClassesLink = document.getElementById("allclasses_navbar_top");
if(window==top) {
allClassesLink.style.display = "block";
}
else {
allClassesLink.style.display = "none";
}
//-->
</script>
</div>
<a name="skip-navbar_top">
<!-- -->
</a></div>
<!-- ========= END OF TOP NAVBAR ========= -->
<div class="header">
<h2 title="Uses of Class com.box.boxjavalibv2.BoxConfigBuilder" class="title">Uses of Class<br>com.box.boxjavalibv2.BoxConfigBuilder</h2>
</div>
<div class="classUseContainer">No usage of com.box.boxjavalibv2.BoxConfigBuilder</div>
<!-- ======= START OF BOTTOM NAVBAR ====== -->
<div class="bottomNav"><a name="navbar_bottom">
<!-- -->
</a><a href="#skip-navbar_bottom" title="Skip navigation links"></a><a name="navbar_bottom_firstrow">
<!-- -->
</a>
<ul class="navList" title="Navigation">
<li><a href="../../../../overview-summary.html">Overview</a></li>
<li><a href="../package-summary.html">Package</a></li>
<li><a href="../../../../com/box/boxjavalibv2/BoxConfigBuilder.html" title="class in com.box.boxjavalibv2">Class</a></li>
<li class="navBarCell1Rev">Use</li>
<li><a href="../../../../overview-tree.html">Tree</a></li>
<li><a href="../../../../deprecated-list.html">Deprecated</a></li>
<li><a href="../../../../index-files/index-1.html">Index</a></li>
<li><a href="../../../../help-doc.html">Help</a></li>
</ul>
</div>
<div class="subNav">
<ul class="navList">
<li>Prev</li>
<li>Next</li>
</ul>
<ul class="navList">
<li><a href="../../../../index.html?com/box/boxjavalibv2/class-use/BoxConfigBuilder.html" target="_top">Frames</a></li>
<li><a href="BoxConfigBuilder.html" target="_top">No Frames</a></li>
</ul>
<ul class="navList" id="allclasses_navbar_bottom">
<li><a href="../../../../allclasses-noframe.html">All Classes</a></li>
</ul>
<div>
<script type="text/javascript"><!--
allClassesLink = document.getElementById("allclasses_navbar_bottom");
if(window==top) {
allClassesLink.style.display = "block";
}
else {
allClassesLink.style.display = "none";
}
//-->
</script>
</div>
<a name="skip-navbar_bottom">
<!-- -->
</a></div>
<!-- ======== END OF BOTTOM NAVBAR ======= -->
</body>
</html>
| {'content_hash': 'd79d4bb0eb14299d739f0cba22fbba68', 'timestamp': '', 'source': 'github', 'line_count': 115, 'max_line_length': 137, 'avg_line_length': 35.88695652173913, 'alnum_prop': 0.619578386236976, 'repo_name': 'shelsonjava/box-java-sdk-v2', 'id': '7caf443b28d63d3702ceace6e8d01a0bf430e345', 'size': '4127', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'javadoc/com/box/boxjavalibv2/class-use/BoxConfigBuilder.html', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Java', 'bytes': '737981'}, {'name': 'Shell', 'bytes': '1413'}]} |
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Title</title>
</head>
<body>
<h1>SHACLEX API</h1>
<ul>
<li><a href="/docs/index.html">API Documentation</a></li>
<li><a href="/dataCheck">Check RDF Data</a></li>
<li><a href="/schemaCheck">Check schemas</a></li>
<li><a href="/validate">Validate RDF Data with a Schema</a></li>
<li><a href="/about.html">About SHACLEX</a></li>
</ul>
</body>
</html> | {'content_hash': 'fe69fea33f09ecee2c6ab948781a6f01', 'timestamp': '', 'source': 'github', 'line_count': 18, 'max_line_length': 68, 'avg_line_length': 26.88888888888889, 'alnum_prop': 0.6053719008264463, 'repo_name': 'labra/apiShaclex', 'id': '17c0bcc6dbaaa915ff2e8a8a94f6a54a9d3ffb06', 'size': '484', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/main/resources/templates/landing.html', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Batchfile', 'bytes': '5127'}, {'name': 'CSS', 'bytes': '21'}, {'name': 'HTML', 'bytes': '3531'}, {'name': 'Java', 'bytes': '24850'}, {'name': 'Shell', 'bytes': '7272'}]} |
class Scene
{
public:
Scene(const std::string& SceneFilePath);
void UnLoad();
void LoadSceneObject(const json& obj, Transform* parent);
bool Load(SharedPtr<World> InWorld);
void LoadCore(json& core);
bool IsNewScene();
void Save(const std::string& fileName, Transform* root);
void SaveSceneRecursively(json& d, Transform* CurrentTransform);
SharedPtr<World> GameWorld;
File CurrentLevel;
Path FilePath;
}; | {'content_hash': 'b48d4b82bab37d2acaba058c264c9234', 'timestamp': '', 'source': 'github', 'line_count': 20, 'max_line_length': 65, 'avg_line_length': 21.05, 'alnum_prop': 0.7482185273159145, 'repo_name': 'wobbier/MitchEngine', 'id': '7d65a01946eff0a6fb26a94a53a1e9ea88ccdea6', 'size': '549', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'Source/World/Scene.h', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Batchfile', 'bytes': '1573'}, {'name': 'C', 'bytes': '3573353'}, {'name': 'C#', 'bytes': '35890'}, {'name': 'C++', 'bytes': '1063321'}, {'name': 'GLSL', 'bytes': '5521'}, {'name': 'HTML', 'bytes': '361'}, {'name': 'Lua', 'bytes': '24016'}, {'name': 'Scala', 'bytes': '269'}, {'name': 'Shell', 'bytes': '34054'}]} |
<?php
// autoload_psr4.php @generated by Composer
$vendorDir = dirname(dirname(__FILE__));
$baseDir = dirname($vendorDir);
return array(
'phpDocumentor\\Reflection\\' => array($vendorDir . '/phpdocumentor/reflection-common/src', $vendorDir . '/phpdocumentor/type-resolver/src', $vendorDir . '/phpdocumentor/reflection-docblock/src'),
'Wn\\Generators\\' => array($vendorDir . '/wn/lumen-generators/src'),
'When\\' => array($vendorDir . '/tplaner/when/src'),
'Webmozart\\Assert\\' => array($vendorDir . '/webmozart/assert/src'),
'TijsVerkoyen\\CssToInlineStyles\\' => array($vendorDir . '/tijsverkoyen/css-to-inline-styles/src'),
'Symfony\\Polyfill\\Mbstring\\' => array($vendorDir . '/symfony/polyfill-mbstring'),
'Symfony\\Component\\Yaml\\' => array($vendorDir . '/symfony/yaml'),
'Symfony\\Component\\Translation\\' => array($vendorDir . '/symfony/translation'),
'Symfony\\Component\\Process\\' => array($vendorDir . '/symfony/process'),
'Symfony\\Component\\HttpKernel\\' => array($vendorDir . '/symfony/http-kernel'),
'Symfony\\Component\\HttpFoundation\\' => array($vendorDir . '/symfony/http-foundation'),
'Symfony\\Component\\Finder\\' => array($vendorDir . '/symfony/finder'),
'Symfony\\Component\\EventDispatcher\\' => array($vendorDir . '/symfony/event-dispatcher'),
'Symfony\\Component\\Debug\\' => array($vendorDir . '/symfony/debug'),
'Symfony\\Component\\CssSelector\\' => array($vendorDir . '/symfony/css-selector'),
'Symfony\\Component\\Console\\' => array($vendorDir . '/symfony/console'),
'SocialiteProviders\\Manager\\Test\\' => array($vendorDir . '/socialiteproviders/manager/tests'),
'SocialiteProviders\\Manager\\' => array($vendorDir . '/socialiteproviders/manager/src'),
'SocialiteProviders\\Google\\' => array($vendorDir . '/socialiteproviders/google'),
'Psr\\Log\\' => array($vendorDir . '/psr/log/Psr/Log'),
'Psr\\Http\\Message\\' => array($vendorDir . '/psr/http-message/src'),
'Monolog\\' => array($vendorDir . '/monolog/monolog/src/Monolog'),
'League\\OAuth1\\' => array($vendorDir . '/league/oauth1-client/src'),
'League\\Flysystem\\AwsS3v3\\' => array($vendorDir . '/league/flysystem-aws-s3-v3/src'),
'League\\Flysystem\\' => array($vendorDir . '/league/flysystem/src'),
'Laravel\\Socialite\\' => array($vendorDir . '/laravel/socialite/src'),
'Laravel\\Lumen\\' => array($vendorDir . '/laravel/lumen-framework/src'),
'JmesPath\\' => array($vendorDir . '/mtdowling/jmespath.php/src'),
'Illuminate\\View\\' => array($vendorDir . '/illuminate/view'),
'Illuminate\\Validation\\' => array($vendorDir . '/illuminate/validation'),
'Illuminate\\Translation\\' => array($vendorDir . '/illuminate/translation'),
'Illuminate\\Support\\' => array($vendorDir . '/illuminate/support'),
'Illuminate\\Session\\' => array($vendorDir . '/illuminate/session'),
'Illuminate\\Queue\\' => array($vendorDir . '/illuminate/queue'),
'Illuminate\\Pipeline\\' => array($vendorDir . '/illuminate/pipeline'),
'Illuminate\\Pagination\\' => array($vendorDir . '/illuminate/pagination'),
'Illuminate\\Mail\\' => array($vendorDir . '/illuminate/mail'),
'Illuminate\\Http\\' => array($vendorDir . '/illuminate/http'),
'Illuminate\\Hashing\\' => array($vendorDir . '/illuminate/hashing'),
'Illuminate\\Filesystem\\' => array($vendorDir . '/illuminate/filesystem'),
'Illuminate\\Events\\' => array($vendorDir . '/illuminate/events'),
'Illuminate\\Encryption\\' => array($vendorDir . '/illuminate/encryption'),
'Illuminate\\Database\\' => array($vendorDir . '/illuminate/database'),
'Illuminate\\Contracts\\' => array($vendorDir . '/illuminate/contracts'),
'Illuminate\\Container\\' => array($vendorDir . '/illuminate/container'),
'Illuminate\\Console\\' => array($vendorDir . '/illuminate/console'),
'Illuminate\\Config\\' => array($vendorDir . '/illuminate/config'),
'Illuminate\\Cache\\' => array($vendorDir . '/illuminate/cache'),
'Illuminate\\Bus\\' => array($vendorDir . '/illuminate/bus'),
'Illuminate\\Broadcasting\\' => array($vendorDir . '/illuminate/broadcasting'),
'Illuminate\\Auth\\' => array($vendorDir . '/illuminate/auth'),
'Ical\\' => array($vendorDir . '/calendar/icsfile/src'),
'Hashids\\' => array($vendorDir . '/hashids/hashids/src'),
'GuzzleHttp\\Psr7\\' => array($vendorDir . '/guzzlehttp/psr7/src'),
'GuzzleHttp\\Promise\\' => array($vendorDir . '/guzzlehttp/promises/src'),
'GuzzleHttp\\' => array($vendorDir . '/guzzlehttp/guzzle/src'),
'FastRoute\\' => array($vendorDir . '/nikic/fast-route/src'),
'Faker\\' => array($vendorDir . '/fzaninotto/faker/src/Faker'),
'Dotenv\\' => array($vendorDir . '/vlucas/phpdotenv/src'),
'Doctrine\\Instantiator\\' => array($vendorDir . '/doctrine/instantiator/src/Doctrine/Instantiator'),
'DeepCopy\\' => array($vendorDir . '/myclabs/deep-copy/src/DeepCopy'),
'Cron\\' => array($vendorDir . '/mtdowling/cron-expression/src/Cron'),
'Collective\\Html\\' => array($vendorDir . '/vluzrmos/collective-html/src'),
'Carbon\\' => array($vendorDir . '/nesbot/carbon/src/Carbon'),
'Aws\\' => array($vendorDir . '/aws/aws-sdk-php/src'),
'App\\' => array($baseDir . '/app'),
);
| {'content_hash': '86c85fdd6d0a7a0aaed7433e343d278d', 'timestamp': '', 'source': 'github', 'line_count': 75, 'max_line_length': 200, 'avg_line_length': 70.53333333333333, 'alnum_prop': 0.6512287334593573, 'repo_name': 'RbnAlexs/GoogleApi', 'id': '9677237eee94d1b777f4ea9f8b8b8932ca681933', 'size': '5290', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'vendor/composer/autoload_psr4.php', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'ApacheConf', 'bytes': '553'}, {'name': 'CSS', 'bytes': '53806'}, {'name': 'HTML', 'bytes': '37402'}, {'name': 'JavaScript', 'bytes': '1616841'}, {'name': 'PHP', 'bytes': '57058'}, {'name': 'Shell', 'bytes': '71'}]} |
//
// This code was auto-generated by AFNOR StoredProcedureSystem, version 1.0
//
using SOURCE.Data.Context;
using SOURCE.Domaine;
using SOURCE.Business.Depots;
using System.Linq;
namespace SOURCE.Data.Depots
{
public partial class DepotLien_Droit_ActionGenerale : Depot<Lien_Droit_ActionGenerale>, IDepotLien_Droit_ActionGenerale
{
/// <summary>
/// Initialise une nouvelle instance du dépôt.
/// </summary>
/// <param name="dataContext">Contexte de la base de donnée.</param>
public DepotLien_Droit_ActionGenerale(ISourceDbContext dataContext)
: base(dataContext)
{
}
}
}
| {'content_hash': 'cd3d63cb0b7bddaa030aa32315cff87e', 'timestamp': '', 'source': 'github', 'line_count': 24, 'max_line_length': 123, 'avg_line_length': 28.5, 'alnum_prop': 0.6491228070175439, 'repo_name': 'apo-j/Projects_Working', 'id': 'c92d9034674d34dae99fa618192f961795398b20', 'size': '1052', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'S/SOURCE/SOURCE.Common/SOURCE.Data/Depots/Generated/DepotLien_Droit_ActionGenerale.cs', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'ASP', 'bytes': '16118'}, {'name': 'Batchfile', 'bytes': '1096'}, {'name': 'C#', 'bytes': '27262375'}, {'name': 'CSS', 'bytes': '8474090'}, {'name': 'Groff', 'bytes': '2101703'}, {'name': 'HTML', 'bytes': '4910101'}, {'name': 'JavaScript', 'bytes': '20716565'}, {'name': 'PHP', 'bytes': '9283'}, {'name': 'XSLT', 'bytes': '930531'}]} |
var ExtensionBase = require('tau/core/extension.base');
module.exports = ExtensionBase.extend({
'bus afterInit': function () {
this.fire('dataBind');
}
});
| {'content_hash': 'e4a690efef4863ec56b714aa801dc34e', 'timestamp': '', 'source': 'github', 'line_count': 7, 'max_line_length': 55, 'avg_line_length': 24.714285714285715, 'alnum_prop': 0.6416184971098265, 'repo_name': 'TargetProcess/tau-tools', 'id': 'bbe1aea892131e3fdb0ca9fd80a6569bbef92766', 'size': '173', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'lib/create/template/component/default/{=instanceName}/extensions/{=instanceName}.extension.js', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'HTML', 'bytes': '63'}, {'name': 'JavaScript', 'bytes': '16835'}]} |
from __future__ import unicode_literals
import frappe
from frappe import _
import frappe.permissions
import re, csv, os
from frappe.utils.csvutils import UnicodeWriter
from frappe.utils import cstr, formatdate, format_datetime, parse_json, cint, format_duration
from frappe.core.doctype.data_import_legacy.importer import get_data_keys
from six import string_types
from frappe.core.doctype.access_log.access_log import make_access_log
reflags = {
"I":re.I,
"L":re.L,
"M":re.M,
"U":re.U,
"S":re.S,
"X":re.X,
"D": re.DEBUG
}
@frappe.whitelist()
def export_data(doctype=None, parent_doctype=None, all_doctypes=True, with_data=False,
select_columns=None, file_type='CSV', template=False, filters=None):
_doctype = doctype
if isinstance(_doctype, list):
_doctype = _doctype[0]
make_access_log(doctype=_doctype, file_type=file_type, columns=select_columns, filters=filters, method=parent_doctype)
exporter = DataExporter(doctype=doctype, parent_doctype=parent_doctype, all_doctypes=all_doctypes, with_data=with_data,
select_columns=select_columns, file_type=file_type, template=template, filters=filters)
exporter.build_response()
class DataExporter:
def __init__(self, doctype=None, parent_doctype=None, all_doctypes=True, with_data=False,
select_columns=None, file_type='CSV', template=False, filters=None):
self.doctype = doctype
self.parent_doctype = parent_doctype
self.all_doctypes = all_doctypes
self.with_data = cint(with_data)
self.select_columns = select_columns
self.file_type = file_type
self.template = template
self.filters = filters
self.data_keys = get_data_keys()
self.prepare_args()
def prepare_args(self):
if self.select_columns:
self.select_columns = parse_json(self.select_columns)
if self.filters:
self.filters = parse_json(self.filters)
self.docs_to_export = {}
if self.doctype:
if isinstance(self.doctype, string_types):
self.doctype = [self.doctype]
if len(self.doctype) > 1:
self.docs_to_export = self.doctype[1]
self.doctype = self.doctype[0]
if not self.parent_doctype:
self.parent_doctype = self.doctype
self.column_start_end = {}
if self.all_doctypes:
self.child_doctypes = []
for df in frappe.get_meta(self.doctype).get_table_fields():
self.child_doctypes.append(dict(doctype=df.options, parentfield=df.fieldname))
def build_response(self):
self.writer = UnicodeWriter()
self.name_field = 'parent' if self.parent_doctype != self.doctype else 'name'
if self.template:
self.add_main_header()
self.writer.writerow([''])
self.tablerow = [self.data_keys.doctype]
self.labelrow = [_("Column Labels:")]
self.fieldrow = [self.data_keys.columns]
self.mandatoryrow = [_("Mandatory:")]
self.typerow = [_('Type:')]
self.inforow = [_('Info:')]
self.columns = []
self.build_field_columns(self.doctype)
if self.all_doctypes:
for d in self.child_doctypes:
self.append_empty_field_column()
if (self.select_columns and self.select_columns.get(d['doctype'], None)) or not self.select_columns:
# if atleast one column is selected for this doctype
self.build_field_columns(d['doctype'], d['parentfield'])
self.add_field_headings()
self.add_data()
if self.with_data and not self.data:
frappe.respond_as_web_page(_('No Data'), _('There is no data to be exported'), indicator_color='orange')
if self.file_type == 'Excel':
self.build_response_as_excel()
else:
# write out response as a type csv
frappe.response['result'] = cstr(self.writer.getvalue())
frappe.response['type'] = 'csv'
frappe.response['doctype'] = self.doctype
def add_main_header(self):
self.writer.writerow([_('Data Import Template')])
self.writer.writerow([self.data_keys.main_table, self.doctype])
if self.parent_doctype != self.doctype:
self.writer.writerow([self.data_keys.parent_table, self.parent_doctype])
else:
self.writer.writerow([''])
self.writer.writerow([''])
self.writer.writerow([_('Notes:')])
self.writer.writerow([_('Please do not change the template headings.')])
self.writer.writerow([_('First data column must be blank.')])
self.writer.writerow([_('If you are uploading new records, leave the "name" (ID) column blank.')])
self.writer.writerow([_('If you are uploading new records, "Naming Series" becomes mandatory, if present.')])
self.writer.writerow([_('Only mandatory fields are necessary for new records. You can delete non-mandatory columns if you wish.')])
self.writer.writerow([_('For updating, you can update only selective columns.')])
self.writer.writerow([_('You can only upload upto 5000 records in one go. (may be less in some cases)')])
if self.name_field == "parent":
self.writer.writerow([_('"Parent" signifies the parent table in which this row must be added')])
self.writer.writerow([_('If you are updating, please select "Overwrite" else existing rows will not be deleted.')])
def build_field_columns(self, dt, parentfield=None):
meta = frappe.get_meta(dt)
# build list of valid docfields
tablecolumns = []
table_name = 'tab' + dt
for f in frappe.db.get_table_columns_description(table_name):
field = meta.get_field(f.name)
if field and ((self.select_columns and f.name in self.select_columns[dt]) or not self.select_columns):
tablecolumns.append(field)
tablecolumns.sort(key = lambda a: int(a.idx))
_column_start_end = frappe._dict(start=0)
if dt==self.doctype:
if (meta.get('autoname') and meta.get('autoname').lower()=='prompt') or (self.with_data):
self._append_name_column()
# if importing only child table for new record, add parent field
if meta.get('istable') and not self.with_data:
self.append_field_column(frappe._dict({
"fieldname": "parent",
"parent": "",
"label": "Parent",
"fieldtype": "Data",
"reqd": 1,
"info": _("Parent is the name of the document to which the data will get added to.")
}), True)
_column_start_end = frappe._dict(start=0)
else:
_column_start_end = frappe._dict(start=len(self.columns))
if self.with_data:
self._append_name_column(dt)
for docfield in tablecolumns:
self.append_field_column(docfield, True)
# all non mandatory fields
for docfield in tablecolumns:
self.append_field_column(docfield, False)
# if there is one column, add a blank column (?)
if len(self.columns)-_column_start_end.start == 1:
self.append_empty_field_column()
# append DocType name
self.tablerow[_column_start_end.start + 1] = dt
if parentfield:
self.tablerow[_column_start_end.start + 2] = parentfield
_column_start_end.end = len(self.columns) + 1
self.column_start_end[(dt, parentfield)] = _column_start_end
def append_field_column(self, docfield, for_mandatory):
if not docfield:
return
if for_mandatory and not docfield.reqd:
return
if not for_mandatory and docfield.reqd:
return
if docfield.fieldname in ('parenttype', 'trash_reason'):
return
if docfield.hidden:
return
if self.select_columns and docfield.fieldname not in self.select_columns.get(docfield.parent, []) \
and docfield.fieldname!="name":
return
self.tablerow.append("")
self.fieldrow.append(docfield.fieldname)
self.labelrow.append(_(docfield.label))
self.mandatoryrow.append(docfield.reqd and 'Yes' or 'No')
self.typerow.append(docfield.fieldtype)
self.inforow.append(self.getinforow(docfield))
self.columns.append(docfield.fieldname)
def append_empty_field_column(self):
self.tablerow.append("~")
self.fieldrow.append("~")
self.labelrow.append("")
self.mandatoryrow.append("")
self.typerow.append("")
self.inforow.append("")
self.columns.append("")
@staticmethod
def getinforow(docfield):
"""make info comment for options, links etc."""
if docfield.fieldtype == 'Select':
if not docfield.options:
return ''
else:
return _("One of") + ': %s' % ', '.join(filter(None, docfield.options.split('\n')))
elif docfield.fieldtype == 'Link':
return 'Valid %s' % docfield.options
elif docfield.fieldtype == 'Int':
return 'Integer'
elif docfield.fieldtype == "Check":
return "0 or 1"
elif docfield.fieldtype in ["Date", "Datetime"]:
return cstr(frappe.defaults.get_defaults().date_format)
elif hasattr(docfield, "info"):
return docfield.info
else:
return ''
def add_field_headings(self):
self.writer.writerow(self.tablerow)
self.writer.writerow(self.labelrow)
self.writer.writerow(self.fieldrow)
self.writer.writerow(self.mandatoryrow)
self.writer.writerow(self.typerow)
self.writer.writerow(self.inforow)
if self.template:
self.writer.writerow([self.data_keys.data_separator])
def add_data(self):
if self.template and not self.with_data:
return
frappe.permissions.can_export(self.parent_doctype, raise_exception=True)
# sort nested set doctypes by `lft asc`
order_by = None
table_columns = frappe.db.get_table_columns(self.parent_doctype)
if 'lft' in table_columns and 'rgt' in table_columns:
order_by = '`tab{doctype}`.`lft` asc'.format(doctype=self.parent_doctype)
# get permitted data only
self.data = frappe.get_list(self.doctype, fields=["*"], filters=self.filters, limit_page_length=None, order_by=order_by)
for doc in self.data:
op = self.docs_to_export.get("op")
names = self.docs_to_export.get("name")
if names and op:
if op == '=' and doc.name not in names:
continue
elif op == '!=' and doc.name in names:
continue
elif names:
try:
sflags = self.docs_to_export.get("flags", "I,U").upper()
flags = 0
for a in re.split('\W+',sflags):
flags = flags | reflags.get(a,0)
c = re.compile(names, flags)
m = c.match(doc.name)
if not m:
continue
except Exception:
if doc.name not in names:
continue
# add main table
rows = []
self.add_data_row(rows, self.doctype, None, doc, 0)
if self.all_doctypes:
# add child tables
for c in self.child_doctypes:
for ci, child in enumerate(frappe.db.sql("""select * from `tab{0}`
where parent=%s and parentfield=%s order by idx""".format(c['doctype']),
(doc.name, c['parentfield']), as_dict=1)):
self.add_data_row(rows, c['doctype'], c['parentfield'], child, ci)
for row in rows:
self.writer.writerow(row)
def add_data_row(self, rows, dt, parentfield, doc, rowidx):
d = doc.copy()
meta = frappe.get_meta(dt)
if self.all_doctypes:
d.name = '"'+ d.name+'"'
if len(rows) < rowidx + 1:
rows.append([""] * (len(self.columns) + 1))
row = rows[rowidx]
_column_start_end = self.column_start_end.get((dt, parentfield))
if _column_start_end:
for i, c in enumerate(self.columns[_column_start_end.start:_column_start_end.end]):
df = meta.get_field(c)
fieldtype = df.fieldtype if df else "Data"
value = d.get(c, "")
if value:
if fieldtype == "Date":
value = formatdate(value)
elif fieldtype == "Datetime":
value = format_datetime(value)
elif fieldtype == "Duration":
value = format_duration(value, df.hide_days)
row[_column_start_end.start + i + 1] = value
def build_response_as_excel(self):
filename = frappe.generate_hash("", 10)
with open(filename, 'wb') as f:
f.write(cstr(self.writer.getvalue()).encode('utf-8'))
f = open(filename)
reader = csv.reader(f)
from frappe.utils.xlsxutils import make_xlsx
xlsx_file = make_xlsx(reader, "Data Import Template" if self.template else 'Data Export')
f.close()
os.remove(filename)
# write out response as a xlsx type
frappe.response['filename'] = self.doctype + '.xlsx'
frappe.response['filecontent'] = xlsx_file.getvalue()
frappe.response['type'] = 'binary'
def _append_name_column(self, dt=None):
self.append_field_column(frappe._dict({
"fieldname": "name" if dt else self.name_field,
"parent": dt or "",
"label": "ID",
"fieldtype": "Data",
"reqd": 1,
}), True)
| {'content_hash': '1746a91e839d8be6f64d11ab63409e01', 'timestamp': '', 'source': 'github', 'line_count': 360, 'max_line_length': 133, 'avg_line_length': 33.13611111111111, 'alnum_prop': 0.685807695531897, 'repo_name': 'adityahase/frappe', 'id': 'bec8cde7eaec888ea6aad4189f31fdd65eedc361', 'size': '12030', 'binary': False, 'copies': '1', 'ref': 'refs/heads/develop', 'path': 'frappe/core/doctype/data_export/exporter.py', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '288806'}, {'name': 'HTML', 'bytes': '209164'}, {'name': 'JavaScript', 'bytes': '2350450'}, {'name': 'Less', 'bytes': '160693'}, {'name': 'Makefile', 'bytes': '99'}, {'name': 'Python', 'bytes': '3035663'}, {'name': 'SCSS', 'bytes': '45340'}, {'name': 'Shell', 'bytes': '517'}, {'name': 'Vue', 'bytes': '73943'}]} |
#include "stdafx.h"
int _tmain(int argc, _TCHAR* argv[])
{
printf("nf1_init.exe [command] [setting]\r\n");
printf("Commands:\r\n");
printf("test [device serial number] - runs bitfury tests to that device\r\n");
printf("fixID [ignored] - Tests for NanoFury devices and fixes Product String\r\n\r\n");
switch(argc){
case 2:
if (wcscmp(argv[1],L"fixID")==0){
printf("fixID mode specified.\r\n\r\n");
spi_init(NULL, L"NanoFury NF1 v0.6");
printf("\r\nfixID mode completed. Please run command again to read new values.\r\n\r\n");
}
case 3:
if (wcscmp(argv[1],L"test")==0){
printf("test mode specified for device %s.\r\n\r\n", argv[2]);
if (spi_init(argv[2], NULL)){
printf("DEVICE FOUND! Proceeding with testing... Press ESC to cancel testing at any time\r\n\r\n", argv[3]);
spitest_main(osc50);
nanofury_device_off(hndNanoFury);
ReleaseMCP2210(hndNanoFury);
}
else{
printf("Device not found. No tests have been performed.\r\n\r\n");
}
}
case 4:
if (wcscmp(argv[1],L"test")==0){
int n = _wtoi(argv[3]);
if ((n<48)||(n>56)) n=48;
printf("test mode specified for device %s with %u bit speed.\r\n\r\n", argv[2], n);
if (spi_init(argv[2], NULL)){
printf("DEVICE FOUND! Proceeding with testing... Press ESC to cancel testing at any time\r\n\r\n", argv[3]);
switch (n){
case 49: spitest_main(osc49); break;
case 50: spitest_main(osc50); break;
case 51: spitest_main(osc51); break;
case 52: spitest_main(osc52); break;
case 53: spitest_main(osc53); break;
case 54: spitest_main(osc54); break;
case 55: spitest_main(osc55); break;
case 56: spitest_main(osc56); break;
default: spitest_main(osc48); break;
}
nanofury_device_off(hndNanoFury);
ReleaseMCP2210(hndNanoFury);
}
else{
printf("Device not found. No tests have been performed.\r\n\r\n");
}
}
break;
default: spi_init(NULL, NULL);
}
//spi_init(true, L"NanoFury NF1 v0.6");
//spi_init(true, NULL);
printf("Press [ENTER] to exit...");
getchar();
return 0;
}
| {'content_hash': '541e1b9c8ee64ad8ca87b12029050c07', 'timestamp': '', 'source': 'github', 'line_count': 71, 'max_line_length': 113, 'avg_line_length': 30.0, 'alnum_prop': 0.6150234741784038, 'repo_name': 'nanofury/NanoFury_Init', 'id': '01759d9f1f91bfde17f3493f8a87c34cac33f385', 'size': '3333', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'nf1_init/nf1_init.cpp', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'C', 'bytes': '328134'}, {'name': 'C++', 'bytes': '39677'}, {'name': 'Objective-C', 'bytes': '1948'}, {'name': 'Shell', 'bytes': '69'}]} |
//Copyright (C) Microsoft Corporation. All rights reserved.
// explicit1.cs
interface IDimensions
{
float Length();
float Width();
}
class Box : IDimensions
{
float lengthInches;
float widthInches;
public Box(float length, float width)
{
lengthInches = length;
widthInches = width;
}
// Explicit interface member implementation:
float IDimensions.Length()
{
return lengthInches;
}
// Explicit interface member implementation:
float IDimensions.Width()
{
return widthInches;
}
public static void Main()
{
// Declare a class instance "myBox":
Box myBox = new Box(30.0f, 20.0f);
// Declare an interface instance "myDimensions":
IDimensions myDimensions = (IDimensions) myBox;
// Print out the dimensions of the box:
/* The following commented lines would produce compilation
errors because they try to access an explicitly implemented
interface member from a class instance: */
//System.Console.WriteLine("Length: {0}", myBox.Length());
//System.Console.WriteLine("Width: {0}", myBox.Width());
/* Print out the dimensions of the box by calling the methods
from an instance of the interface: */
System.Console.WriteLine("Length: {0}", myDimensions.Length());
System.Console.WriteLine("Width: {0}", myDimensions.Width());
}
}
| {'content_hash': 'd7d0dcd34b473b161081036126adfe61', 'timestamp': '', 'source': 'github', 'line_count': 49, 'max_line_length': 70, 'avg_line_length': 29.714285714285715, 'alnum_prop': 0.6318681318681318, 'repo_name': 'SiddharthMishraPersonal/StudyMaterials', 'id': '6d88b805a3e4f9e47db7bf0d829772051e7882ca', 'size': '1458', 'binary': False, 'copies': '2', 'ref': 'refs/heads/master', 'path': 'Linq/CSharpSamples/LanguageSamples/ExplicitInterface/ExplicitInterface1/explicit1.cs', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'C', 'bytes': '1'}, {'name': 'C#', 'bytes': '1963252'}, {'name': 'C++', 'bytes': '7401'}, {'name': 'CSS', 'bytes': '31609'}, {'name': 'Java', 'bytes': '14635'}, {'name': 'Objective-C', 'bytes': '368454'}]} |
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<!-- NewPage -->
<html lang="pt">
<head>
<!-- Generated by javadoc (version 1.7.0_79) on Mon Jun 22 15:50:37 BRT 2015 -->
<title>Uses of Class net.floodlightcontroller.packet.BPDU</title>
<meta name="date" content="2015-06-22">
<link rel="stylesheet" type="text/css" href="../../../../stylesheet.css" title="Style">
</head>
<body>
<script type="text/javascript"><!--
if (location.href.indexOf('is-external=true') == -1) {
parent.document.title="Uses of Class net.floodlightcontroller.packet.BPDU";
}
//-->
</script>
<noscript>
<div>JavaScript is disabled on your browser.</div>
</noscript>
<!-- ========= START OF TOP NAVBAR ======= -->
<div class="topNav"><a name="navbar_top">
<!-- -->
</a><a href="#skip-navbar_top" title="Skip navigation links"></a><a name="navbar_top_firstrow">
<!-- -->
</a>
<ul class="navList" title="Navigation">
<li><a href="../../../../overview-summary.html">Overview</a></li>
<li><a href="../package-summary.html">Package</a></li>
<li><a href="../../../../net/floodlightcontroller/packet/BPDU.html" title="class in net.floodlightcontroller.packet">Class</a></li>
<li class="navBarCell1Rev">Use</li>
<li><a href="../package-tree.html">Tree</a></li>
<li><a href="../../../../deprecated-list.html">Deprecated</a></li>
<li><a href="../../../../index-files/index-1.html">Index</a></li>
<li><a href="../../../../help-doc.html">Help</a></li>
</ul>
</div>
<div class="subNav">
<ul class="navList">
<li>Prev</li>
<li>Next</li>
</ul>
<ul class="navList">
<li><a href="../../../../index.html?net/floodlightcontroller/packet/class-use/BPDU.html" target="_top">Frames</a></li>
<li><a href="BPDU.html" target="_top">No Frames</a></li>
</ul>
<ul class="navList" id="allclasses_navbar_top">
<li><a href="../../../../allclasses-noframe.html">All Classes</a></li>
</ul>
<div>
<script type="text/javascript"><!--
allClassesLink = document.getElementById("allclasses_navbar_top");
if(window==top) {
allClassesLink.style.display = "block";
}
else {
allClassesLink.style.display = "none";
}
//-->
</script>
</div>
<a name="skip-navbar_top">
<!-- -->
</a></div>
<!-- ========= END OF TOP NAVBAR ========= -->
<div class="header">
<h2 title="Uses of Class net.floodlightcontroller.packet.BPDU" class="title">Uses of Class<br>net.floodlightcontroller.packet.BPDU</h2>
</div>
<div class="classUseContainer">No usage of net.floodlightcontroller.packet.BPDU</div>
<!-- ======= START OF BOTTOM NAVBAR ====== -->
<div class="bottomNav"><a name="navbar_bottom">
<!-- -->
</a><a href="#skip-navbar_bottom" title="Skip navigation links"></a><a name="navbar_bottom_firstrow">
<!-- -->
</a>
<ul class="navList" title="Navigation">
<li><a href="../../../../overview-summary.html">Overview</a></li>
<li><a href="../package-summary.html">Package</a></li>
<li><a href="../../../../net/floodlightcontroller/packet/BPDU.html" title="class in net.floodlightcontroller.packet">Class</a></li>
<li class="navBarCell1Rev">Use</li>
<li><a href="../package-tree.html">Tree</a></li>
<li><a href="../../../../deprecated-list.html">Deprecated</a></li>
<li><a href="../../../../index-files/index-1.html">Index</a></li>
<li><a href="../../../../help-doc.html">Help</a></li>
</ul>
</div>
<div class="subNav">
<ul class="navList">
<li>Prev</li>
<li>Next</li>
</ul>
<ul class="navList">
<li><a href="../../../../index.html?net/floodlightcontroller/packet/class-use/BPDU.html" target="_top">Frames</a></li>
<li><a href="BPDU.html" target="_top">No Frames</a></li>
</ul>
<ul class="navList" id="allclasses_navbar_bottom">
<li><a href="../../../../allclasses-noframe.html">All Classes</a></li>
</ul>
<div>
<script type="text/javascript"><!--
allClassesLink = document.getElementById("allclasses_navbar_bottom");
if(window==top) {
allClassesLink.style.display = "block";
}
else {
allClassesLink.style.display = "none";
}
//-->
</script>
</div>
<a name="skip-navbar_bottom">
<!-- -->
</a></div>
<!-- ======== END OF BOTTOM NAVBAR ======= -->
</body>
</html>
| {'content_hash': '0b51d7c574d8f01bd992795bd4bcaeb3', 'timestamp': '', 'source': 'github', 'line_count': 115, 'max_line_length': 135, 'avg_line_length': 35.61739130434783, 'alnum_prop': 0.62109375, 'repo_name': 'paulorvj/sdnvoip', 'id': 'c7c40b4705e20d93d66ae5785c646971dc9ad125', 'size': '4096', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'doc/net/floodlightcontroller/packet/class-use/BPDU.html', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'CSS', 'bytes': '181'}, {'name': 'HTML', 'bytes': '10186'}, {'name': 'Java', 'bytes': '3772566'}, {'name': 'JavaScript', 'bytes': '55112'}, {'name': 'Makefile', 'bytes': '426'}, {'name': 'Python', 'bytes': '32743'}, {'name': 'Shell', 'bytes': '5610'}, {'name': 'Thrift', 'bytes': '7114'}]} |
asp-classic-cms
===============
ASP classic and SQL based Content Management System
I first built this CMS in 2007 for a client. I have since moved to Wordpress for the CMS now, so I'm making this CMS available free to use. If you have questions, Google my name and get in touch.
| {'content_hash': '38d92e395e394112e899084b110933e2', 'timestamp': '', 'source': 'github', 'line_count': 6, 'max_line_length': 195, 'avg_line_length': 47.0, 'alnum_prop': 0.7340425531914894, 'repo_name': 'mayurjobanputra/asp-classic-cms', 'id': '13a9a15be5ab33805b2c9e5050ab7428febdb417', 'size': '282', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'README.md', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'ASP', 'bytes': '25368'}, {'name': 'JavaScript', 'bytes': '8397'}]} |
"""Main command line interface for fm_server."""
| {'content_hash': '5f2727069f7bfbcff6521c54fec062ab', 'timestamp': '', 'source': 'github', 'line_count': 1, 'max_line_length': 48, 'avg_line_length': 49.0, 'alnum_prop': 0.7142857142857143, 'repo_name': 'nstoik/farm_monitor', 'id': '0f0d5a4d526083d45dd8c1e91cca2b0988a38cbd', 'size': '49', 'binary': False, 'copies': '1', 'ref': 'refs/heads/main', 'path': 'server/fm_server/cli/__init__.py', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Dockerfile', 'bytes': '18259'}, {'name': 'HCL', 'bytes': '1534'}, {'name': 'HTML', 'bytes': '611'}, {'name': 'JavaScript', 'bytes': '268'}, {'name': 'Mako', 'bytes': '494'}, {'name': 'Python', 'bytes': '242717'}, {'name': 'Shell', 'bytes': '2937'}, {'name': 'TypeScript', 'bytes': '18970'}, {'name': 'Vue', 'bytes': '14394'}]} |
yii2-widget-sidenav
===================
[](https://packagist.org/packages/kartik-v/yii2-widget-sidenav)
[](https://packagist.org/packages/kartik-v/yii2-widget-sidenav)
[](https://packagist.org/packages/kartik-v/yii2-widget-sidenav)
[](https://packagist.org/packages/kartik-v/yii2-widget-sidenav)
[](https://packagist.org/packages/kartik-v/yii2-widget-sidenav)
This widget is a collapsible side navigation menu built to seamlessly work with Bootstrap framework. It is built over Bootstrap [stacked nav](http://getbootstrap.com/components/#nav-pills) component. This widget class extends the [Yii Menu widget](https://github.com/yiisoft/yii2/blob/master/framework/widgets/Menu.php). Upto 3 levels of submenus are by default supported by the CSS styles to balance performance and useability. You can choose to extend it to more or less levels by customizing the [CSS](https://github.com/kartik-v/yii2-widgets/blob/master/assets/css/sidenav.css).
> NOTE: This extension is a sub repo split of [yii2-widgets](https://github.com/kartik-v/yii2-widgets). The split has been done since 08-Nov-2014 to allow developers to install this specific widget in isolation if needed. One can also use the extension the previous way with the whole suite of [yii2-widgets](http://demos.krajee.com/widgets).
## Installation
The preferred way to install this extension is through [composer](http://getcomposer.org/download/). Check the [composer.json](https://github.com/kartik-v/yii2-widget-sidenav/blob/master/composer.json) for this extension's requirements and dependencies. Read this [web tip /wiki](http://webtips.krajee.com/setting-composer-minimum-stability-application/) on setting the `minimum-stability` settings for your application's composer.json.
To install, either run
```
$ php composer.phar require kartik-v/yii2-widget-sidenav "*"
```
or add
```
"kartik-v/yii2-widget-sidenav": "*"
```
to the ```require``` section of your `composer.json` file.
## Latest Release
> NOTE: The latest version of the module is v1.0.0 released on 08-Nov-2014. Refer the [CHANGE LOG](https://github.com/kartik-v/yii2-widget-sidenav/blob/master/CHANGE.md) for details.
## Demo
You can refer detailed [documentation and demos](http://demos.krajee.com/widget-details/sidenav) on usage of the extension.
## Usage
```php
use kartik\sidenav\SideNav;
echo SideNav::widget([
'type' => SideNav::TYPE_DEFAULT,
'heading' => 'Options',
'items' => [
[
'url' => '#',
'label' => 'Home',
'icon' => 'home'
],
[
'label' => 'Help',
'icon' => 'question-sign',
'items' => [
['label' => 'About', 'icon'=>'info-sign', 'url'=>'#'],
['label' => 'Contact', 'icon'=>'phone', 'url'=>'#'],
],
],
],
]);
```
## License
**yii2-widget-sidenav** is released under the BSD 3-Clause License. See the bundled `LICENSE.md` for details. | {'content_hash': '3a720d40cebac6054a3def0f59281f29', 'timestamp': '', 'source': 'github', 'line_count': 68, 'max_line_length': 583, 'avg_line_length': 47.911764705882355, 'alnum_prop': 0.7228360957642725, 'repo_name': 'aaesis/brdsdev', 'id': 'cfe4698dcdb793d908ac9c82a97ffcf3e21d2f6e', 'size': '3258', 'binary': False, 'copies': '2', 'ref': 'refs/heads/master', 'path': 'vendor/kartik-v/yii2-widget-sidenav/README.md', 'mode': '33188', 'license': 'bsd-3-clause', 'language': [{'name': 'ApacheConf', 'bytes': '112'}, {'name': 'Batchfile', 'bytes': '1041'}, {'name': 'CSS', 'bytes': '48678'}, {'name': 'JavaScript', 'bytes': '55220'}, {'name': 'PHP', 'bytes': '447086'}]} |
struct WebApplicationInfo;
namespace content {
struct LoadCommittedDetails;
}
namespace extensions {
class Extension;
class LocationBarController;
class ScriptBadgeController;
class ScriptBubbleController;
// Per-tab extension helper. Also handles non-extension apps.
class TabHelper : public content::WebContentsObserver,
public ExtensionFunctionDispatcher::Delegate,
public ImageLoadingTracker::Observer,
public AppNotifyChannelSetup::Delegate,
public base::SupportsWeakPtr<TabHelper>,
public content::NotificationObserver,
public content::WebContentsUserData<TabHelper> {
public:
// Different types of action when web app info is available.
// OnDidGetApplicationInfo uses this to dispatch calls.
enum WebAppAction {
NONE, // No action at all.
CREATE_SHORTCUT, // Bring up create application shortcut dialog.
UPDATE_SHORTCUT // Update icon for app shortcut.
};
// Observer base class for classes listening for content script messages
// from the renderer.
class ContentScriptObserver {
public:
// Map of extensions IDs to the executing script paths.
typedef std::map<std::string, std::set<std::string> > ExecutingScriptsMap;
// Automatically observes and unobserves |tab_helper| on construction
// and destruction. |tab_helper| must outlive |this|.
explicit ContentScriptObserver(TabHelper* tab_helper);
ContentScriptObserver();
virtual void OnContentScriptsExecuting(
const content::WebContents* web_contents,
const ExecutingScriptsMap& executing_scripts_map,
int32 on_page_id,
const GURL& on_url) = 0;
protected:
virtual ~ContentScriptObserver();
TabHelper* tab_helper_;
};
virtual ~TabHelper();
void AddContentScriptObserver(ContentScriptObserver* observer) {
content_script_observers_.AddObserver(observer);
}
void RemoveContentScriptObserver(ContentScriptObserver* observer) {
content_script_observers_.RemoveObserver(observer);
}
void CreateApplicationShortcuts();
bool CanCreateApplicationShortcuts() const;
void set_pending_web_app_action(WebAppAction action) {
pending_web_app_action_ = action;
}
// App extensions ------------------------------------------------------------
// Sets the extension denoting this as an app. If |extension| is non-null this
// tab becomes an app-tab. WebContents does not listen for unload events for
// the extension. It's up to consumers of WebContents to do that.
//
// NOTE: this should only be manipulated before the tab is added to a browser.
// TODO(sky): resolve if this is the right way to identify an app tab. If it
// is, than this should be passed in the constructor.
void SetExtensionApp(const Extension* extension);
// Convenience for setting the app extension by id. This does nothing if
// |extension_app_id| is empty, or an extension can't be found given the
// specified id.
void SetExtensionAppById(const std::string& extension_app_id);
// Set just the app icon, used by panels created by an extension.
void SetExtensionAppIconById(const std::string& extension_app_id);
const Extension* extension_app() const { return extension_app_; }
bool is_app() const { return extension_app_ != NULL; }
const WebApplicationInfo& web_app_info() const {
return web_app_info_;
}
// If an app extension has been explicitly set for this WebContents its icon
// is returned.
//
// NOTE: the returned icon is larger than 16x16 (its size is
// Extension::EXTENSION_ICON_SMALLISH).
SkBitmap* GetExtensionAppIcon();
content::WebContents* web_contents() const {
return content::WebContentsObserver::web_contents();
}
ScriptExecutor* script_executor() {
return &script_executor_;
}
LocationBarController* location_bar_controller() {
return location_bar_controller_.get();
}
ActiveTabPermissionGranter* active_tab_permission_granter() {
return active_tab_permission_granter_.get();
}
ScriptBubbleController* script_bubble_controller() {
return script_bubble_controller_.get();
}
// Sets a non-extension app icon associated with WebContents and fires an
// INVALIDATE_TYPE_TITLE navigation state change to trigger repaint of title.
void SetAppIcon(const SkBitmap& app_icon);
private:
explicit TabHelper(content::WebContents* web_contents);
friend class content::WebContentsUserData<TabHelper>;
// content::WebContentsObserver overrides.
virtual void RenderViewCreated(
content::RenderViewHost* render_view_host) OVERRIDE;
virtual void DidNavigateMainFrame(
const content::LoadCommittedDetails& details,
const content::FrameNavigateParams& params) OVERRIDE;
virtual bool OnMessageReceived(const IPC::Message& message) OVERRIDE;
virtual void DidCloneToNewWebContents(
content::WebContents* old_web_contents,
content::WebContents* new_web_contents) OVERRIDE;
// ExtensionFunctionDispatcher::Delegate overrides.
virtual extensions::WindowController* GetExtensionWindowController()
const OVERRIDE;
virtual content::WebContents* GetAssociatedWebContents() const OVERRIDE;
// Message handlers.
void OnDidGetApplicationInfo(int32 page_id, const WebApplicationInfo& info);
void OnInstallApplication(const WebApplicationInfo& info);
void OnInlineWebstoreInstall(int install_id,
int return_route_id,
const std::string& webstore_item_id,
const GURL& requestor_url);
void OnGetAppNotifyChannel(const GURL& requestor_url,
const std::string& client_id,
int return_route_id,
int callback_id);
void OnGetAppInstallState(const GURL& requestor_url,
int return_route_id,
int callback_id);
void OnRequest(const ExtensionHostMsg_Request_Params& params);
void OnContentScriptsExecuting(
const ContentScriptObserver::ExecutingScriptsMap& extension_ids,
int32 page_id,
const GURL& on_url);
// App extensions related methods:
// Resets app_icon_ and if |extension| is non-null creates a new
// ImageLoadingTracker to load the extension's image.
void UpdateExtensionAppIcon(const Extension* extension);
const Extension* GetExtension(
const std::string& extension_app_id);
// ImageLoadingTracker::Observer.
virtual void OnImageLoaded(const gfx::Image& image,
const std::string& extension_id,
int index) OVERRIDE;
// WebstoreStandaloneInstaller::Callback.
virtual void OnInlineInstallComplete(int install_id,
int return_route_id,
bool success,
const std::string& error);
// AppNotifyChannelSetup::Delegate.
virtual void AppNotifyChannelSetupComplete(
const std::string& channel_id,
const std::string& error,
const AppNotifyChannelSetup* setup) OVERRIDE;
// content::NotificationObserver.
virtual void Observe(int type,
const content::NotificationSource& source,
const content::NotificationDetails& details) OVERRIDE;
// Requests application info for the specified page. This is an asynchronous
// request. The delegate is notified by way of OnDidGetApplicationInfo when
// the data is available.
void GetApplicationInfo(int32 page_id);
// Data for app extensions ---------------------------------------------------
// Our content script observers. Declare at top so that it will outlive all
// other members, since they might add themselves as observers.
ObserverList<ContentScriptObserver> content_script_observers_;
// If non-null this tab is an app tab and this is the extension the tab was
// created for.
const Extension* extension_app_;
// Icon for extension_app_ (if non-null) or a manually-set icon for
// non-extension apps.
SkBitmap extension_app_icon_;
// Process any extension messages coming from the tab.
ExtensionFunctionDispatcher extension_function_dispatcher_;
// Used for loading extension_app_icon_.
scoped_ptr<ImageLoadingTracker> extension_app_image_loader_;
// Cached web app info data.
WebApplicationInfo web_app_info_;
// Which deferred action to perform when OnDidGetApplicationInfo is notified
// from a WebContents.
WebAppAction pending_web_app_action_;
content::NotificationRegistrar registrar_;
ScriptExecutor script_executor_;
scoped_ptr<LocationBarController> location_bar_controller_;
scoped_ptr<ActiveTabPermissionGranter> active_tab_permission_granter_;
scoped_ptr<ScriptBubbleController> script_bubble_controller_;
DISALLOW_COPY_AND_ASSIGN(TabHelper);
};
} // namespace extensions
#endif // CHROME_BROWSER_EXTENSIONS_TAB_HELPER_H_
| {'content_hash': '7dfb142555823d9cac76d1aac4501339', 'timestamp': '', 'source': 'github', 'line_count': 245, 'max_line_length': 80, 'avg_line_length': 36.90204081632653, 'alnum_prop': 0.694945249419312, 'repo_name': 'junmin-zhu/chromium-rivertrail', 'id': '5d8708e794eb90839c6b291e6f9f75d1dac1e7f5', 'size': '10052', 'binary': False, 'copies': '1', 'ref': 'refs/heads/v8-binding', 'path': 'chrome/browser/extensions/tab_helper.h', 'mode': '33188', 'license': 'bsd-3-clause', 'language': [{'name': 'ASP', 'bytes': '853'}, {'name': 'AppleScript', 'bytes': '6973'}, {'name': 'Arduino', 'bytes': '464'}, {'name': 'Assembly', 'bytes': '1172794'}, {'name': 'Awk', 'bytes': '9519'}, {'name': 'C', 'bytes': '75806807'}, {'name': 'C#', 'bytes': '1132'}, {'name': 'C++', 'bytes': '145161929'}, {'name': 'DOT', 'bytes': '1559'}, {'name': 'F#', 'bytes': '381'}, {'name': 'Java', 'bytes': '1546515'}, {'name': 'JavaScript', 'bytes': '18675242'}, {'name': 'Logos', 'bytes': '4517'}, {'name': 'Matlab', 'bytes': '5234'}, {'name': 'Objective-C', 'bytes': '6981387'}, {'name': 'PHP', 'bytes': '97817'}, {'name': 'Perl', 'bytes': '926245'}, {'name': 'Python', 'bytes': '8088373'}, {'name': 'R', 'bytes': '262'}, {'name': 'Ragel in Ruby Host', 'bytes': '3239'}, {'name': 'Shell', 'bytes': '1513486'}, {'name': 'Tcl', 'bytes': '277077'}, {'name': 'XML', 'bytes': '13493'}]} |
void SystemInit(void)
{
return;
}
| {'content_hash': 'bf1fee85e67006d537e73af12c21cf5b', 'timestamp': '', 'source': 'github', 'line_count': 4, 'max_line_length': 21, 'avg_line_length': 9.5, 'alnum_prop': 0.631578947368421, 'repo_name': 'ekohandel/AM335x', 'id': 'b7fb27ca5d4a223907238ebe495577a0c3bb76cb', 'size': '38', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/systeminit.c', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Assembly', 'bytes': '6062'}, {'name': 'C', 'bytes': '109'}, {'name': 'Makefile', 'bytes': '276'}]} |
<?php
namespace AppBundle\Repository;
/**
* RateRepository.
*
* This class was generated by the Doctrine ORM. Add your own custom
* repository methods below.
*/
class RateRepository extends \Doctrine\ORM\EntityRepository
{
public function getActiveRates()
{
return $this
->createQueryBuilder('r')
->where('r.active = true')
->getQuery()
->getResult();
}
}
| {'content_hash': '250c2fbab52725ba802381eb04305770', 'timestamp': '', 'source': 'github', 'line_count': 21, 'max_line_length': 68, 'avg_line_length': 20.523809523809526, 'alnum_prop': 0.6102088167053364, 'repo_name': 'alexseif/myapp', 'id': '24da315b6901b02236ee10f9d46b9117dbb57759', 'size': '431', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/AppBundle/Repository/RateRepository.php', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '144422'}, {'name': 'HTML', 'bytes': '32801'}, {'name': 'JavaScript', 'bytes': '113706'}, {'name': 'Less', 'bytes': '201281'}, {'name': 'PHP', 'bytes': '512370'}, {'name': 'SCSS', 'bytes': '221642'}, {'name': 'Shell', 'bytes': '2824'}, {'name': 'Twig', 'bytes': '611941'}]} |
package dlp.snippets;
// [START dlp_deidentify_table_row_suppress]
import com.google.cloud.dlp.v2.DlpServiceClient;
import com.google.privacy.dlp.v2.ContentItem;
import com.google.privacy.dlp.v2.DeidentifyConfig;
import com.google.privacy.dlp.v2.DeidentifyContentRequest;
import com.google.privacy.dlp.v2.DeidentifyContentResponse;
import com.google.privacy.dlp.v2.FieldId;
import com.google.privacy.dlp.v2.LocationName;
import com.google.privacy.dlp.v2.RecordCondition;
import com.google.privacy.dlp.v2.RecordCondition.Condition;
import com.google.privacy.dlp.v2.RecordCondition.Conditions;
import com.google.privacy.dlp.v2.RecordCondition.Expressions;
import com.google.privacy.dlp.v2.RecordSuppression;
import com.google.privacy.dlp.v2.RecordTransformations;
import com.google.privacy.dlp.v2.RelationalOperator;
import com.google.privacy.dlp.v2.Table;
import com.google.privacy.dlp.v2.Table.Row;
import com.google.privacy.dlp.v2.Value;
import java.io.IOException;
public class DeIdentifyTableRowSuppress {
public static void deIdentifyTableRowSuppress() throws IOException {
// TODO(developer): Replace these variables before running the sample.
String projectId = "your-project-id";
Table tableToDeIdentify =
Table.newBuilder()
.addHeaders(FieldId.newBuilder().setName("AGE").build())
.addHeaders(FieldId.newBuilder().setName("PATIENT").build())
.addHeaders(FieldId.newBuilder().setName("HAPPINESS SCORE").build())
.addRows(
Row.newBuilder()
.addValues(Value.newBuilder().setStringValue("101").build())
.addValues(Value.newBuilder().setStringValue("Charles Dickens").build())
.addValues(Value.newBuilder().setStringValue("95").build())
.build())
.addRows(
Row.newBuilder()
.addValues(Value.newBuilder().setStringValue("22").build())
.addValues(Value.newBuilder().setStringValue("Jane Austen").build())
.addValues(Value.newBuilder().setStringValue("21").build())
.build())
.addRows(
Row.newBuilder()
.addValues(Value.newBuilder().setStringValue("55").build())
.addValues(Value.newBuilder().setStringValue("Mark Twain").build())
.addValues(Value.newBuilder().setStringValue("75").build())
.build())
.build();
deIdentifyTableRowSuppress(projectId, tableToDeIdentify);
}
public static Table deIdentifyTableRowSuppress(String projectId, Table tableToDeIdentify)
throws IOException {
// Initialize client that will be used to send requests. This client only needs to be created
// once, and can be reused for multiple requests. After completing all of your requests, call
// the "close" method on the client to safely clean up any remaining background resources.
try (DlpServiceClient dlp = DlpServiceClient.create()) {
// Specify what content you want the service to de-identify.
ContentItem contentItem = ContentItem.newBuilder().setTable(tableToDeIdentify).build();
// Specify when the content should be de-identified.
Condition condition =
Condition.newBuilder()
.setField(FieldId.newBuilder().setName("AGE").build())
.setOperator(RelationalOperator.GREATER_THAN)
.setValue(Value.newBuilder().setIntegerValue(89).build())
.build();
// Apply the condition to record suppression.
RecordSuppression recordSuppressions =
RecordSuppression.newBuilder()
.setCondition(
RecordCondition.newBuilder()
.setExpressions(
Expressions.newBuilder()
.setConditions(
Conditions.newBuilder().addConditions(condition).build())
.build())
.build())
.build();
// Use record suppression as the only transformation
RecordTransformations transformations =
RecordTransformations.newBuilder().addRecordSuppressions(recordSuppressions).build();
DeidentifyConfig deidentifyConfig =
DeidentifyConfig.newBuilder().setRecordTransformations(transformations).build();
// Combine configurations into a request for the service.
DeidentifyContentRequest request =
DeidentifyContentRequest.newBuilder()
.setParent(LocationName.of(projectId, "global").toString())
.setItem(contentItem)
.setDeidentifyConfig(deidentifyConfig)
.build();
// Send the request and receive response from the service.
DeidentifyContentResponse response = dlp.deidentifyContent(request);
// Print the results.
System.out.println("Table after de-identification: " + response.getItem().getTable());
return response.getItem().getTable();
}
}
}
// [END dlp_deidentify_table_row_suppress]
| {'content_hash': '0640074cb822b1cc55c3a284d1c0cf54', 'timestamp': '', 'source': 'github', 'line_count': 112, 'max_line_length': 97, 'avg_line_length': 45.839285714285715, 'alnum_prop': 0.6583560576548501, 'repo_name': 'googleapis/java-dlp', 'id': '0c02dc1664967c0fd52d78b953477ce77ad99be5', 'size': '5723', 'binary': False, 'copies': '1', 'ref': 'refs/heads/main', 'path': 'samples/snippets/src/main/java/dlp/snippets/DeIdentifyTableRowSuppress.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Batchfile', 'bytes': '801'}, {'name': 'Java', 'bytes': '12264243'}, {'name': 'Python', 'bytes': '2548'}, {'name': 'Shell', 'bytes': '22242'}]} |
set -o errexit
set -o nounset
set -o pipefail
REPO_ROOT="$(cd "$(dirname "${BASH_SOURCE}")/.." && pwd -P)"
reindex_out="$(${REPO_ROOT}/scripts/reindex.sh)"
if [[ -n "${reindex_out}" ]]; then
echo "Invalid index: file name matches directory name" >&2
echo "${reindex_out}" >&2
echo "Please run 'scripts/reindex.sh'" >&2
exit 1
fi
| {'content_hash': '75d5f6215b3bfd0e8ccb2fafed37f0a6', 'timestamp': '', 'source': 'github', 'line_count': 13, 'max_line_length': 60, 'avg_line_length': 26.076923076923077, 'alnum_prop': 0.6283185840707964, 'repo_name': 'dcos/dcos-docs', 'id': 'cf200e0250d93f48df062d44a4f84147b21c0654', 'size': '360', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'scripts/validate-reindex.sh', 'mode': '33261', 'license': 'apache-2.0', 'language': [{'name': 'HTML', 'bytes': '172997'}, {'name': 'Makefile', 'bytes': '28'}, {'name': 'Shell', 'bytes': '30642'}]} |
from django.core.urlresolvers import reverse
from django.views.generic import ListView, CreateView, UpdateView, DeleteView, DetailView
from contacts.models import Address, Contact
import forms
#The ListView that we subclass from is itself composed of several mixins that provide some behaviour !!!
class ListContactView(ListView):
model = Contact # this view is going to list all the Contacts in our database
template_name = 'contact_list.html'
# Most generic views that do form processing have
# the concept of the 'success URL': where to redirect
# the user when the form is successfully submitted.
class CreateContactView(CreateView):
model = Contact
template_name = 'edit_contact.html'
# after adding 'email_filed' in forms.py we have to tell this view to use it
form_class = forms.ContactForm
def get_success_url(self):
return reverse('contacts-list') # contact_list - the name of a view defined in URLS !
# in order to use the same template for create and update (add/edit) we have to add info
# about where the form should redirect to the context
def get_context_data(self, **kwargs):
context = super(CreateContactView, self).get_context_data(**kwargs)
context['action'] = reverse('contacts-new')
return context
class UpdateContactView(UpdateView):
model = Contact
template_name = 'edit_contact.html'
# after adding 'email_filed' in forms.py we have to tell this view to use it
form_class = forms.ContactForm
def get_success_url(self):
return reverse('contacts-list')
# in order to use the same template for create and update (add/edit) we have to add info
# about where the form should redirect to the context
def get_context_data(self, **kwargs):
context = super(UpdateContactView, self).get_context_data(**kwargs)
context['action'] = reverse('contacts-edit', kwargs = {'pk': self.get_object().id})
return context
class DeleteContactView(DeleteView):
model = Contact
template_name = 'delete_contact.html'
def get_success_url(self):
return reverse('contacts-list')
class ContactView(DetailView):
model = Contact
template_name = 'contact.html'
def get_context_data(self, **kwargs):
context = super(ContactView, self).get_context_data(**kwargs)
home_addresses = Address.objects.filter(contact_id=self.get_object().id, address_type='HOME')
context['home_address'] = home_addresses[0] if home_addresses else None
return context
#
# to work with Contact and Address models together
#
# class EditContactAddressView(UpdateView):
# model = Contact # inline formset takes the parent object as its starting point
# template_name = 'edit_addresses.html'
# form_class = forms.ContactAddressFormSet
# def get_success_url(self):
# # redirect to the Contact view.
# return self.get_object().get_absolute_url()
class EditContactAddressView(UpdateView):
model = Address # inline formset takes the parent object as its starting point
template_name = 'edit_addresses.html'
#form_class = forms.ContactAddressFormSet
def get_success_url(self):
return reverse('contacts-list')
def get_context_data(self, **kwargs):
context = super(EditContactAddressView, self).get_context_data(**kwargs)
context['action'] = reverse('contacts-edit-addresses', kwargs = {'pk': self.get_object().id})
return context | {'content_hash': 'f00c2500cff048bedb2466680547d367', 'timestamp': '', 'source': 'github', 'line_count': 107, 'max_line_length': 109, 'avg_line_length': 33.42056074766355, 'alnum_prop': 0.6868008948545862, 'repo_name': 'pyjosh/django_addressbook', 'id': 'cf9811648e3c79af51e38ddbceef984ad6716568', 'size': '3602', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'contacts/views.py', 'mode': '33188', 'license': 'mit', 'language': []} |
require 'rubygems'
require 'priority_queue'
class String
# Convenience method so we can iterate over characters easily
def to_chars
a = []
each_byte do |b|
a << b.chr
end
a
end
end
module TileMap
# Although start is a plains, its cost is 0
START = 0
PLAINS = 1
FOREST = 2
MOUNTAIN = 3
WATER = nil
class TilePath < Array
def initialize(map)
@map = map
super([@map.start])
end
def cost
inject(0) {|sum, c| sum + @map.tile(*c) }
end
end
class Map
attr_reader :start, :goal
# parse a string contining a tile map into a nested array
def initialize(map_str)
@tiles = []
map_str.each do |line|
@tiles << []
line.chomp.to_chars.each do |c|
@tiles.last << case c
when "@"
START
when ".", "X"
PLAINS
when "*"
FOREST
when "^"
MOUNTAIN
when "~"
WATER
else
raise "Invalid tile type"
end
if '@' == c
@start = [@tiles.last.length - 1, @tiles.length - 1]
elsif 'X' == c
@goal = [@tiles.last.length - 1, @tiles.length - 1]
end
end
end
unless @start && @goal
raise "Either position or goal tile are not set"
end
end
def tile(x, y)
@tiles[y][x]
end
def move_choices(x, y)
if tile(x, y) == WATER
raise "Illegal tile"
end
choices = []
(-1..1).each do |i|
ypos = y + i
if ypos >= 0 && ypos < @tiles.length
(-1..1).each do |j|
xpos = x + j
if xpos >= 0 && xpos < @tiles[i].length
new_position = [xpos, ypos]
if new_position != [x, y] && tile(*new_position) != WATER
choices << new_position
end
end
end
end
end
choices
end
def self.manhattan(point1, point2)
((point2[0] - point1[0]) + (point2[1] - point1[1])).abs
end
end
def self.a_star_search(map)
# To store points we have already visited, so we don't repeat ourselves
closed = []
open = PriorityQueue.new
# Start off the queue with one path, which will contain the start position
open.push TilePath.new(map), 0
while ! open.empty?
# Get the path with the best cost to expand
current_path = open.delete_min_return_key
pos = current_path.last
unless closed.include?(pos)
if pos == map.goal
return current_path
end
closed << pos
# Create new paths and add them to the priority queue
map.move_choices(*pos).each do |p|
heuristic = Map.manhattan(p, map.goal)
new_path = current_path.clone << p
open.push(new_path, new_path.cost + heuristic)
end
end
end
raise "Cannot be solved"
end
end
@m = TileMap::Map.new(File.read('large_map.txt'))
results = TileMap.a_star_search(@m)
puts results.map! {|pos| pos.join(",") }.join(" ")
| {'content_hash': '66d92164bb4e682f43505bf198360530', 'timestamp': '', 'source': 'github', 'line_count': 135, 'max_line_length': 78, 'avg_line_length': 24.444444444444443, 'alnum_prop': 0.4890909090909091, 'repo_name': 'J-Y/RubyQuiz', 'id': '8cc84457ded88669aae7d9c865553113aa3e87f4', 'size': '3300', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'ruby_quiz/quiz98_sols/solutions/Roland Swingler/a_star.rb', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'ApacheConf', 'bytes': '2281'}, {'name': 'Bison', 'bytes': '261'}, {'name': 'C', 'bytes': '23577'}, {'name': 'CSS', 'bytes': '10128'}, {'name': 'GAP', 'bytes': '4684'}, {'name': 'HTML', 'bytes': '186387'}, {'name': 'JavaScript', 'bytes': '148'}, {'name': 'Makefile', 'bytes': '6175'}, {'name': 'OCaml', 'bytes': '7884'}, {'name': 'Ruby', 'bytes': '8196401'}, {'name': 'Shell', 'bytes': '1092'}, {'name': 'TeX', 'bytes': '4601'}]} |
// See https://i3wm.org/docs/ipc.html for protocol information
#define _POSIX_C_SOURCE 200112L
#include <linux/input-event-codes.h>
#include <assert.h>
#include <errno.h>
#include <fcntl.h>
#include <json.h>
#include <stdbool.h>
#include <stdint.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/socket.h>
#include <sys/ioctl.h>
#include <sys/un.h>
#include <unistd.h>
#include <wayland-server-core.h>
#include "sway/commands.h"
#include "sway/config.h"
#include "sway/desktop/transaction.h"
#include "sway/ipc-json.h"
#include "sway/ipc-server.h"
#include "sway/output.h"
#include "sway/server.h"
#include "sway/input/input-manager.h"
#include "sway/input/keyboard.h"
#include "sway/input/seat.h"
#include "sway/tree/root.h"
#include "sway/tree/view.h"
#include "sway/tree/workspace.h"
#include "list.h"
#include "log.h"
#include "util.h"
static int ipc_socket = -1;
static struct wl_event_source *ipc_event_source = NULL;
static struct sockaddr_un *ipc_sockaddr = NULL;
static list_t *ipc_client_list = NULL;
static struct wl_listener ipc_display_destroy;
static const char ipc_magic[] = {'i', '3', '-', 'i', 'p', 'c'};
#define IPC_HEADER_SIZE (sizeof(ipc_magic) + 8)
struct ipc_client {
struct wl_event_source *event_source;
struct wl_event_source *writable_event_source;
struct sway_server *server;
int fd;
enum ipc_command_type subscribed_events;
size_t write_buffer_len;
size_t write_buffer_size;
char *write_buffer;
// The following are for storing data between event_loop calls
uint32_t pending_length;
enum ipc_command_type pending_type;
};
struct sockaddr_un *ipc_user_sockaddr(void);
int ipc_handle_connection(int fd, uint32_t mask, void *data);
int ipc_client_handle_readable(int client_fd, uint32_t mask, void *data);
int ipc_client_handle_writable(int client_fd, uint32_t mask, void *data);
void ipc_client_disconnect(struct ipc_client *client);
void ipc_client_handle_command(struct ipc_client *client, uint32_t payload_length,
enum ipc_command_type payload_type);
bool ipc_send_reply(struct ipc_client *client, enum ipc_command_type payload_type,
const char *payload, uint32_t payload_length);
static void handle_display_destroy(struct wl_listener *listener, void *data) {
if (ipc_event_source) {
wl_event_source_remove(ipc_event_source);
}
close(ipc_socket);
unlink(ipc_sockaddr->sun_path);
while (ipc_client_list->length) {
ipc_client_disconnect(ipc_client_list->items[ipc_client_list->length-1]);
}
list_free(ipc_client_list);
free(ipc_sockaddr);
wl_list_remove(&ipc_display_destroy.link);
}
void ipc_init(struct sway_server *server) {
ipc_socket = socket(AF_UNIX, SOCK_STREAM, 0);
if (ipc_socket == -1) {
sway_abort("Unable to create IPC socket");
}
if (fcntl(ipc_socket, F_SETFD, FD_CLOEXEC) == -1) {
sway_abort("Unable to set CLOEXEC on IPC socket");
}
if (fcntl(ipc_socket, F_SETFL, O_NONBLOCK) == -1) {
sway_abort("Unable to set NONBLOCK on IPC socket");
}
ipc_sockaddr = ipc_user_sockaddr();
// We want to use socket name set by user, not existing socket from another sway instance.
if (getenv("SWAYSOCK") != NULL && access(getenv("SWAYSOCK"), F_OK) == -1) {
strncpy(ipc_sockaddr->sun_path, getenv("SWAYSOCK"), sizeof(ipc_sockaddr->sun_path) - 1);
ipc_sockaddr->sun_path[sizeof(ipc_sockaddr->sun_path) - 1] = 0;
}
unlink(ipc_sockaddr->sun_path);
if (bind(ipc_socket, (struct sockaddr *)ipc_sockaddr, sizeof(*ipc_sockaddr)) == -1) {
sway_abort("Unable to bind IPC socket");
}
if (listen(ipc_socket, 3) == -1) {
sway_abort("Unable to listen on IPC socket");
}
// Set i3 IPC socket path so that i3-msg works out of the box
setenv("I3SOCK", ipc_sockaddr->sun_path, 1);
setenv("SWAYSOCK", ipc_sockaddr->sun_path, 1);
ipc_client_list = create_list();
ipc_display_destroy.notify = handle_display_destroy;
wl_display_add_destroy_listener(server->wl_display, &ipc_display_destroy);
ipc_event_source = wl_event_loop_add_fd(server->wl_event_loop, ipc_socket,
WL_EVENT_READABLE, ipc_handle_connection, server);
}
struct sockaddr_un *ipc_user_sockaddr(void) {
struct sockaddr_un *ipc_sockaddr = malloc(sizeof(struct sockaddr_un));
if (ipc_sockaddr == NULL) {
sway_abort("Can't allocate ipc_sockaddr");
}
ipc_sockaddr->sun_family = AF_UNIX;
int path_size = sizeof(ipc_sockaddr->sun_path);
// Env var typically set by logind, e.g. "/run/user/<user-id>"
const char *dir = getenv("XDG_RUNTIME_DIR");
if (!dir) {
dir = "/tmp";
}
if (path_size <= snprintf(ipc_sockaddr->sun_path, path_size,
"%s/sway-ipc.%u.%i.sock", dir, getuid(), getpid())) {
sway_abort("Socket path won't fit into ipc_sockaddr->sun_path");
}
return ipc_sockaddr;
}
int ipc_handle_connection(int fd, uint32_t mask, void *data) {
(void) fd;
struct sway_server *server = data;
sway_log(SWAY_DEBUG, "Event on IPC listening socket");
assert(mask == WL_EVENT_READABLE);
int client_fd = accept(ipc_socket, NULL, NULL);
if (client_fd == -1) {
sway_log_errno(SWAY_ERROR, "Unable to accept IPC client connection");
return 0;
}
int flags;
if ((flags = fcntl(client_fd, F_GETFD)) == -1
|| fcntl(client_fd, F_SETFD, flags|FD_CLOEXEC) == -1) {
sway_log_errno(SWAY_ERROR, "Unable to set CLOEXEC on IPC client socket");
close(client_fd);
return 0;
}
if ((flags = fcntl(client_fd, F_GETFL)) == -1
|| fcntl(client_fd, F_SETFL, flags|O_NONBLOCK) == -1) {
sway_log_errno(SWAY_ERROR, "Unable to set NONBLOCK on IPC client socket");
close(client_fd);
return 0;
}
struct ipc_client *client = malloc(sizeof(struct ipc_client));
if (!client) {
sway_log(SWAY_ERROR, "Unable to allocate ipc client");
close(client_fd);
return 0;
}
client->server = server;
client->pending_length = 0;
client->fd = client_fd;
client->subscribed_events = 0;
client->event_source = wl_event_loop_add_fd(server->wl_event_loop,
client_fd, WL_EVENT_READABLE, ipc_client_handle_readable, client);
client->writable_event_source = NULL;
client->write_buffer_size = 128;
client->write_buffer_len = 0;
client->write_buffer = malloc(client->write_buffer_size);
if (!client->write_buffer) {
sway_log(SWAY_ERROR, "Unable to allocate ipc client write buffer");
close(client_fd);
return 0;
}
sway_log(SWAY_DEBUG, "New client: fd %d", client_fd);
list_add(ipc_client_list, client);
return 0;
}
int ipc_client_handle_readable(int client_fd, uint32_t mask, void *data) {
struct ipc_client *client = data;
if (mask & WL_EVENT_ERROR) {
sway_log(SWAY_ERROR, "IPC Client socket error, removing client");
ipc_client_disconnect(client);
return 0;
}
if (mask & WL_EVENT_HANGUP) {
sway_log(SWAY_DEBUG, "Client %d hung up", client->fd);
ipc_client_disconnect(client);
return 0;
}
sway_log(SWAY_DEBUG, "Client %d readable", client->fd);
int read_available;
if (ioctl(client_fd, FIONREAD, &read_available) == -1) {
sway_log_errno(SWAY_INFO, "Unable to read IPC socket buffer size");
ipc_client_disconnect(client);
return 0;
}
// Wait for the rest of the command payload in case the header has already been read
if (client->pending_length > 0) {
if ((uint32_t)read_available >= client->pending_length) {
// Reset pending values.
uint32_t pending_length = client->pending_length;
enum ipc_command_type pending_type = client->pending_type;
client->pending_length = 0;
ipc_client_handle_command(client, pending_length, pending_type);
}
return 0;
}
if (read_available < (int) IPC_HEADER_SIZE) {
return 0;
}
uint8_t buf[IPC_HEADER_SIZE];
// Should be fully available, because read_available >= IPC_HEADER_SIZE
ssize_t received = recv(client_fd, buf, IPC_HEADER_SIZE, 0);
if (received == -1) {
sway_log_errno(SWAY_INFO, "Unable to receive header from IPC client");
ipc_client_disconnect(client);
return 0;
}
if (memcmp(buf, ipc_magic, sizeof(ipc_magic)) != 0) {
sway_log(SWAY_DEBUG, "IPC header check failed");
ipc_client_disconnect(client);
return 0;
}
memcpy(&client->pending_length, buf + sizeof(ipc_magic), sizeof(uint32_t));
memcpy(&client->pending_type, buf + sizeof(ipc_magic) + sizeof(uint32_t), sizeof(uint32_t));
if (read_available - received >= (long)client->pending_length) {
// Reset pending values.
uint32_t pending_length = client->pending_length;
enum ipc_command_type pending_type = client->pending_type;
client->pending_length = 0;
ipc_client_handle_command(client, pending_length, pending_type);
}
return 0;
}
static bool ipc_has_event_listeners(enum ipc_command_type event) {
for (int i = 0; i < ipc_client_list->length; i++) {
struct ipc_client *client = ipc_client_list->items[i];
if ((client->subscribed_events & event_mask(event)) != 0) {
return true;
}
}
return false;
}
static void ipc_send_event(const char *json_string, enum ipc_command_type event) {
struct ipc_client *client;
for (int i = 0; i < ipc_client_list->length; i++) {
client = ipc_client_list->items[i];
if ((client->subscribed_events & event_mask(event)) == 0) {
continue;
}
if (!ipc_send_reply(client, event, json_string,
(uint32_t)strlen(json_string))) {
sway_log_errno(SWAY_INFO, "Unable to send reply to IPC client");
/* ipc_send_reply destroys client on error, which also
* removes it from the list, so we need to process
* current index again */
i--;
}
}
}
void ipc_event_workspace(struct sway_workspace *old,
struct sway_workspace *new, const char *change) {
if (!ipc_has_event_listeners(IPC_EVENT_WORKSPACE)) {
return;
}
sway_log(SWAY_DEBUG, "Sending workspace::%s event", change);
json_object *obj = json_object_new_object();
json_object_object_add(obj, "change", json_object_new_string(change));
if (old) {
json_object_object_add(obj, "old",
ipc_json_describe_node_recursive(&old->node));
} else {
json_object_object_add(obj, "old", NULL);
}
if (new) {
json_object_object_add(obj, "current",
ipc_json_describe_node_recursive(&new->node));
} else {
json_object_object_add(obj, "current", NULL);
}
const char *json_string = json_object_to_json_string(obj);
ipc_send_event(json_string, IPC_EVENT_WORKSPACE);
json_object_put(obj);
}
void ipc_event_window(struct sway_container *window, const char *change) {
if (!ipc_has_event_listeners(IPC_EVENT_WINDOW)) {
return;
}
sway_log(SWAY_DEBUG, "Sending window::%s event", change);
json_object *obj = json_object_new_object();
json_object_object_add(obj, "change", json_object_new_string(change));
json_object_object_add(obj, "container",
ipc_json_describe_node_recursive(&window->node));
const char *json_string = json_object_to_json_string(obj);
ipc_send_event(json_string, IPC_EVENT_WINDOW);
json_object_put(obj);
}
void ipc_event_barconfig_update(struct bar_config *bar) {
if (!ipc_has_event_listeners(IPC_EVENT_BARCONFIG_UPDATE)) {
return;
}
sway_log(SWAY_DEBUG, "Sending barconfig_update event");
json_object *json = ipc_json_describe_bar_config(bar);
const char *json_string = json_object_to_json_string(json);
ipc_send_event(json_string, IPC_EVENT_BARCONFIG_UPDATE);
json_object_put(json);
}
void ipc_event_bar_state_update(struct bar_config *bar) {
if (!ipc_has_event_listeners(IPC_EVENT_BAR_STATE_UPDATE)) {
return;
}
sway_log(SWAY_DEBUG, "Sending bar_state_update event");
json_object *json = json_object_new_object();
json_object_object_add(json, "id", json_object_new_string(bar->id));
json_object_object_add(json, "visible_by_modifier",
json_object_new_boolean(bar->visible_by_modifier));
const char *json_string = json_object_to_json_string(json);
ipc_send_event(json_string, IPC_EVENT_BAR_STATE_UPDATE);
json_object_put(json);
}
void ipc_event_mode(const char *mode, bool pango) {
if (!ipc_has_event_listeners(IPC_EVENT_MODE)) {
return;
}
sway_log(SWAY_DEBUG, "Sending mode::%s event", mode);
json_object *obj = json_object_new_object();
json_object_object_add(obj, "change", json_object_new_string(mode));
json_object_object_add(obj, "pango_markup",
json_object_new_boolean(pango));
const char *json_string = json_object_to_json_string(obj);
ipc_send_event(json_string, IPC_EVENT_MODE);
json_object_put(obj);
}
void ipc_event_shutdown(const char *reason) {
if (!ipc_has_event_listeners(IPC_EVENT_SHUTDOWN)) {
return;
}
sway_log(SWAY_DEBUG, "Sending shutdown::%s event", reason);
json_object *json = json_object_new_object();
json_object_object_add(json, "change", json_object_new_string(reason));
const char *json_string = json_object_to_json_string(json);
ipc_send_event(json_string, IPC_EVENT_SHUTDOWN);
json_object_put(json);
}
void ipc_event_binding(struct sway_binding *binding) {
if (!ipc_has_event_listeners(IPC_EVENT_BINDING)) {
return;
}
sway_log(SWAY_DEBUG, "Sending binding event");
json_object *json_binding = json_object_new_object();
json_object_object_add(json_binding, "command", json_object_new_string(binding->command));
const char *names[10];
int len = get_modifier_names(names, binding->modifiers);
json_object *modifiers = json_object_new_array();
for (int i = 0; i < len; ++i) {
json_object_array_add(modifiers, json_object_new_string(names[i]));
}
json_object_object_add(json_binding, "event_state_mask", modifiers);
json_object *input_codes = json_object_new_array();
int input_code = 0;
json_object *symbols = json_object_new_array();
json_object *symbol = NULL;
switch (binding->type) {
case BINDING_KEYCODE:; // bindcode: populate input_codes
uint32_t keycode;
for (int i = 0; i < binding->keys->length; ++i) {
keycode = *(uint32_t *)binding->keys->items[i];
json_object_array_add(input_codes, json_object_new_int(keycode));
if (i == 0) {
input_code = keycode;
}
}
break;
case BINDING_KEYSYM:
case BINDING_MOUSESYM:
case BINDING_MOUSECODE:; // bindsym/mouse: populate symbols
uint32_t keysym;
char buffer[64];
for (int i = 0; i < binding->keys->length; ++i) {
keysym = *(uint32_t *)binding->keys->items[i];
if (keysym >= BTN_LEFT && keysym <= BTN_LEFT + 8) {
snprintf(buffer, 64, "button%u", keysym - BTN_LEFT + 1);
} else if (xkb_keysym_get_name(keysym, buffer, 64) < 0) {
continue;
}
json_object *str = json_object_new_string(buffer);
if (i == 0) {
// str is owned by both symbol and symbols. Make sure
// to bump the ref count.
json_object_array_add(symbols, json_object_get(str));
symbol = str;
} else {
json_object_array_add(symbols, str);
}
}
break;
default:
sway_log(SWAY_DEBUG, "Unsupported ipc binding event");
json_object_put(input_codes);
json_object_put(symbols);
json_object_put(json_binding);
return; // do not send any event
}
json_object_object_add(json_binding, "input_codes", input_codes);
json_object_object_add(json_binding, "input_code", json_object_new_int(input_code));
json_object_object_add(json_binding, "symbols", symbols);
json_object_object_add(json_binding, "symbol", symbol);
bool mouse = binding->type == BINDING_MOUSECODE ||
binding->type == BINDING_MOUSESYM;
json_object_object_add(json_binding, "input_type", mouse
? json_object_new_string("mouse")
: json_object_new_string("keyboard"));
json_object *json = json_object_new_object();
json_object_object_add(json, "change", json_object_new_string("run"));
json_object_object_add(json, "binding", json_binding);
const char *json_string = json_object_to_json_string(json);
ipc_send_event(json_string, IPC_EVENT_BINDING);
json_object_put(json);
}
static void ipc_event_tick(const char *payload) {
if (!ipc_has_event_listeners(IPC_EVENT_TICK)) {
return;
}
sway_log(SWAY_DEBUG, "Sending tick event");
json_object *json = json_object_new_object();
json_object_object_add(json, "first", json_object_new_boolean(false));
json_object_object_add(json, "payload", json_object_new_string(payload));
const char *json_string = json_object_to_json_string(json);
ipc_send_event(json_string, IPC_EVENT_TICK);
json_object_put(json);
}
void ipc_event_input(const char *change, struct sway_input_device *device) {
if (!ipc_has_event_listeners(IPC_EVENT_INPUT)) {
return;
}
sway_log(SWAY_DEBUG, "Sending input event");
json_object *json = json_object_new_object();
json_object_object_add(json, "change", json_object_new_string(change));
json_object_object_add(json, "input", ipc_json_describe_input(device));
const char *json_string = json_object_to_json_string(json);
ipc_send_event(json_string, IPC_EVENT_INPUT);
json_object_put(json);
}
int ipc_client_handle_writable(int client_fd, uint32_t mask, void *data) {
struct ipc_client *client = data;
if (mask & WL_EVENT_ERROR) {
sway_log(SWAY_ERROR, "IPC Client socket error, removing client");
ipc_client_disconnect(client);
return 0;
}
if (mask & WL_EVENT_HANGUP) {
sway_log(SWAY_DEBUG, "Client %d hung up", client->fd);
ipc_client_disconnect(client);
return 0;
}
if (client->write_buffer_len <= 0) {
return 0;
}
sway_log(SWAY_DEBUG, "Client %d writable", client->fd);
ssize_t written = write(client->fd, client->write_buffer, client->write_buffer_len);
if (written == -1 && errno == EAGAIN) {
return 0;
} else if (written == -1) {
sway_log_errno(SWAY_INFO, "Unable to send data from queue to IPC client");
ipc_client_disconnect(client);
return 0;
}
memmove(client->write_buffer, client->write_buffer + written, client->write_buffer_len - written);
client->write_buffer_len -= written;
if (client->write_buffer_len == 0 && client->writable_event_source) {
wl_event_source_remove(client->writable_event_source);
client->writable_event_source = NULL;
}
return 0;
}
void ipc_client_disconnect(struct ipc_client *client) {
if (!sway_assert(client != NULL, "client != NULL")) {
return;
}
shutdown(client->fd, SHUT_RDWR);
sway_log(SWAY_INFO, "IPC Client %d disconnected", client->fd);
wl_event_source_remove(client->event_source);
if (client->writable_event_source) {
wl_event_source_remove(client->writable_event_source);
}
int i = 0;
while (i < ipc_client_list->length && ipc_client_list->items[i] != client) {
i++;
}
list_del(ipc_client_list, i);
free(client->write_buffer);
close(client->fd);
free(client);
}
static void ipc_get_workspaces_callback(struct sway_workspace *workspace,
void *data) {
json_object *workspace_json = ipc_json_describe_node(&workspace->node);
// override the default focused indicator because
// it's set differently for the get_workspaces reply
struct sway_seat *seat = input_manager_get_default_seat();
struct sway_workspace *focused_ws = seat_get_focused_workspace(seat);
bool focused = workspace == focused_ws;
json_object_object_del(workspace_json, "focused");
json_object_object_add(workspace_json, "focused",
json_object_new_boolean(focused));
json_object_array_add((json_object *)data, workspace_json);
focused_ws = output_get_active_workspace(workspace->output);
bool visible = workspace == focused_ws;
json_object_object_add(workspace_json, "visible",
json_object_new_boolean(visible));
}
static void ipc_get_marks_callback(struct sway_container *con, void *data) {
json_object *marks = (json_object *)data;
for (int i = 0; i < con->marks->length; ++i) {
char *mark = (char *)con->marks->items[i];
json_object_array_add(marks, json_object_new_string(mark));
}
}
void ipc_client_handle_command(struct ipc_client *client, uint32_t payload_length,
enum ipc_command_type payload_type) {
if (!sway_assert(client != NULL, "client != NULL")) {
return;
}
char *buf = malloc(payload_length + 1);
if (!buf) {
sway_log_errno(SWAY_INFO, "Unable to allocate IPC payload");
ipc_client_disconnect(client);
return;
}
if (payload_length > 0) {
// Payload should be fully available
ssize_t received = recv(client->fd, buf, payload_length, 0);
if (received == -1)
{
sway_log_errno(SWAY_INFO, "Unable to receive payload from IPC client");
ipc_client_disconnect(client);
free(buf);
return;
}
}
buf[payload_length] = '\0';
switch (payload_type) {
case IPC_COMMAND:
{
char *line = strtok(buf, "\n");
while (line) {
size_t line_length = strlen(line);
if (line + line_length >= buf + payload_length) {
break;
}
line[line_length] = ';';
line = strtok(NULL, "\n");
}
list_t *res_list = execute_command(buf, NULL, NULL);
transaction_commit_dirty();
char *json = cmd_results_to_json(res_list);
int length = strlen(json);
ipc_send_reply(client, payload_type, json, (uint32_t)length);
free(json);
while (res_list->length) {
struct cmd_results *results = res_list->items[0];
free_cmd_results(results);
list_del(res_list, 0);
}
list_free(res_list);
goto exit_cleanup;
}
case IPC_SEND_TICK:
{
ipc_event_tick(buf);
ipc_send_reply(client, payload_type, "{\"success\": true}", 17);
goto exit_cleanup;
}
case IPC_GET_OUTPUTS:
{
json_object *outputs = json_object_new_array();
for (int i = 0; i < root->outputs->length; ++i) {
struct sway_output *output = root->outputs->items[i];
json_object *output_json = ipc_json_describe_node(&output->node);
// override the default focused indicator because it's set
// differently for the get_outputs reply
struct sway_seat *seat = input_manager_get_default_seat();
struct sway_workspace *focused_ws =
seat_get_focused_workspace(seat);
bool focused = focused_ws && output == focused_ws->output;
json_object_object_del(output_json, "focused");
json_object_object_add(output_json, "focused",
json_object_new_boolean(focused));
const char *subpixel = sway_wl_output_subpixel_to_string(output->wlr_output->subpixel);
json_object_object_add(output_json, "subpixel_hinting", json_object_new_string(subpixel));
json_object_array_add(outputs, output_json);
}
struct sway_output *output;
wl_list_for_each(output, &root->all_outputs, link) {
if (!output->enabled && output != root->fallback_output) {
json_object_array_add(outputs,
ipc_json_describe_disabled_output(output));
}
}
const char *json_string = json_object_to_json_string(outputs);
ipc_send_reply(client, payload_type, json_string,
(uint32_t)strlen(json_string));
json_object_put(outputs); // free
goto exit_cleanup;
}
case IPC_GET_WORKSPACES:
{
json_object *workspaces = json_object_new_array();
root_for_each_workspace(ipc_get_workspaces_callback, workspaces);
const char *json_string = json_object_to_json_string(workspaces);
ipc_send_reply(client, payload_type, json_string,
(uint32_t)strlen(json_string));
json_object_put(workspaces); // free
goto exit_cleanup;
}
case IPC_SUBSCRIBE:
{
// TODO: Check if they're permitted to use these events
struct json_object *request = json_tokener_parse(buf);
if (request == NULL || !json_object_is_type(request, json_type_array)) {
const char msg[] = "{\"success\": false}";
ipc_send_reply(client, payload_type, msg, strlen(msg));
sway_log(SWAY_INFO, "Failed to parse subscribe request");
goto exit_cleanup;
}
bool is_tick = false;
// parse requested event types
for (size_t i = 0; i < json_object_array_length(request); i++) {
const char *event_type = json_object_get_string(json_object_array_get_idx(request, i));
if (strcmp(event_type, "workspace") == 0) {
client->subscribed_events |= event_mask(IPC_EVENT_WORKSPACE);
} else if (strcmp(event_type, "barconfig_update") == 0) {
client->subscribed_events |= event_mask(IPC_EVENT_BARCONFIG_UPDATE);
} else if (strcmp(event_type, "bar_state_update") == 0) {
client->subscribed_events |= event_mask(IPC_EVENT_BAR_STATE_UPDATE);
} else if (strcmp(event_type, "mode") == 0) {
client->subscribed_events |= event_mask(IPC_EVENT_MODE);
} else if (strcmp(event_type, "shutdown") == 0) {
client->subscribed_events |= event_mask(IPC_EVENT_SHUTDOWN);
} else if (strcmp(event_type, "window") == 0) {
client->subscribed_events |= event_mask(IPC_EVENT_WINDOW);
} else if (strcmp(event_type, "binding") == 0) {
client->subscribed_events |= event_mask(IPC_EVENT_BINDING);
} else if (strcmp(event_type, "tick") == 0) {
client->subscribed_events |= event_mask(IPC_EVENT_TICK);
is_tick = true;
} else if (strcmp(event_type, "input") == 0) {
client->subscribed_events |= event_mask(IPC_EVENT_INPUT);
} else {
const char msg[] = "{\"success\": false}";
ipc_send_reply(client, payload_type, msg, strlen(msg));
json_object_put(request);
sway_log(SWAY_INFO, "Unsupported event type in subscribe request");
goto exit_cleanup;
}
}
json_object_put(request);
const char msg[] = "{\"success\": true}";
ipc_send_reply(client, payload_type, msg, strlen(msg));
if (is_tick) {
const char tickmsg[] = "{\"first\": true, \"payload\": \"\"}";
ipc_send_reply(client, IPC_EVENT_TICK, tickmsg,
strlen(tickmsg));
}
goto exit_cleanup;
}
case IPC_GET_INPUTS:
{
json_object *inputs = json_object_new_array();
struct sway_input_device *device = NULL;
wl_list_for_each(device, &server.input->devices, link) {
json_object_array_add(inputs, ipc_json_describe_input(device));
}
const char *json_string = json_object_to_json_string(inputs);
ipc_send_reply(client, payload_type, json_string,
(uint32_t)strlen(json_string));
json_object_put(inputs); // free
goto exit_cleanup;
}
case IPC_GET_SEATS:
{
json_object *seats = json_object_new_array();
struct sway_seat *seat = NULL;
wl_list_for_each(seat, &server.input->seats, link) {
json_object_array_add(seats, ipc_json_describe_seat(seat));
}
const char *json_string = json_object_to_json_string(seats);
ipc_send_reply(client, payload_type, json_string,
(uint32_t)strlen(json_string));
json_object_put(seats); // free
goto exit_cleanup;
}
case IPC_GET_TREE:
{
json_object *tree = ipc_json_describe_node_recursive(&root->node);
const char *json_string = json_object_to_json_string(tree);
ipc_send_reply(client, payload_type, json_string,
(uint32_t)strlen(json_string));
json_object_put(tree);
goto exit_cleanup;
}
case IPC_GET_MARKS:
{
json_object *marks = json_object_new_array();
root_for_each_container(ipc_get_marks_callback, marks);
const char *json_string = json_object_to_json_string(marks);
ipc_send_reply(client, payload_type, json_string,
(uint32_t)strlen(json_string));
json_object_put(marks);
goto exit_cleanup;
}
case IPC_GET_VERSION:
{
json_object *version = ipc_json_get_version();
const char *json_string = json_object_to_json_string(version);
ipc_send_reply(client, payload_type, json_string,
(uint32_t)strlen(json_string));
json_object_put(version); // free
goto exit_cleanup;
}
case IPC_GET_BAR_CONFIG:
{
if (!buf[0]) {
// Send list of configured bar IDs
json_object *bars = json_object_new_array();
for (int i = 0; i < config->bars->length; ++i) {
struct bar_config *bar = config->bars->items[i];
json_object_array_add(bars, json_object_new_string(bar->id));
}
const char *json_string = json_object_to_json_string(bars);
ipc_send_reply(client, payload_type, json_string,
(uint32_t)strlen(json_string));
json_object_put(bars); // free
} else {
// Send particular bar's details
struct bar_config *bar = NULL;
for (int i = 0; i < config->bars->length; ++i) {
bar = config->bars->items[i];
if (strcmp(buf, bar->id) == 0) {
break;
}
bar = NULL;
}
if (!bar) {
const char *error = "{ \"success\": false, \"error\": \"No bar with that ID\" }";
ipc_send_reply(client, payload_type, error,
(uint32_t)strlen(error));
goto exit_cleanup;
}
json_object *json = ipc_json_describe_bar_config(bar);
const char *json_string = json_object_to_json_string(json);
ipc_send_reply(client, payload_type, json_string,
(uint32_t)strlen(json_string));
json_object_put(json); // free
}
goto exit_cleanup;
}
case IPC_GET_BINDING_MODES:
{
json_object *modes = json_object_new_array();
for (int i = 0; i < config->modes->length; i++) {
struct sway_mode *mode = config->modes->items[i];
json_object_array_add(modes, json_object_new_string(mode->name));
}
const char *json_string = json_object_to_json_string(modes);
ipc_send_reply(client, payload_type, json_string,
(uint32_t)strlen(json_string));
json_object_put(modes); // free
goto exit_cleanup;
}
case IPC_GET_BINDING_STATE:
{
json_object *current_mode = ipc_json_get_binding_mode();
const char *json_string = json_object_to_json_string(current_mode);
ipc_send_reply(client, payload_type, json_string,
(uint32_t)strlen(json_string));
json_object_put(current_mode); // free
goto exit_cleanup;
}
case IPC_GET_CONFIG:
{
json_object *json = json_object_new_object();
json_object_object_add(json, "config", json_object_new_string(config->current_config));
const char *json_string = json_object_to_json_string(json);
ipc_send_reply(client, payload_type, json_string,
(uint32_t)strlen(json_string));
json_object_put(json); // free
goto exit_cleanup;
}
case IPC_SYNC:
{
// It was decided sway will not support this, just return success:false
const char msg[] = "{\"success\": false}";
ipc_send_reply(client, payload_type, msg, strlen(msg));
goto exit_cleanup;
}
default:
sway_log(SWAY_INFO, "Unknown IPC command type %x", payload_type);
goto exit_cleanup;
}
exit_cleanup:
free(buf);
return;
}
bool ipc_send_reply(struct ipc_client *client, enum ipc_command_type payload_type,
const char *payload, uint32_t payload_length) {
assert(payload);
char data[IPC_HEADER_SIZE];
memcpy(data, ipc_magic, sizeof(ipc_magic));
memcpy(data + sizeof(ipc_magic), &payload_length, sizeof(payload_length));
memcpy(data + sizeof(ipc_magic) + sizeof(payload_length), &payload_type, sizeof(payload_type));
while (client->write_buffer_len + IPC_HEADER_SIZE + payload_length >=
client->write_buffer_size) {
client->write_buffer_size *= 2;
}
if (client->write_buffer_size > 4e6) { // 4 MB
sway_log(SWAY_ERROR, "Client write buffer too big (%zu), disconnecting client",
client->write_buffer_size);
ipc_client_disconnect(client);
return false;
}
char *new_buffer = realloc(client->write_buffer, client->write_buffer_size);
if (!new_buffer) {
sway_log(SWAY_ERROR, "Unable to reallocate ipc client write buffer");
ipc_client_disconnect(client);
return false;
}
client->write_buffer = new_buffer;
memcpy(client->write_buffer + client->write_buffer_len, data, IPC_HEADER_SIZE);
client->write_buffer_len += IPC_HEADER_SIZE;
memcpy(client->write_buffer + client->write_buffer_len, payload, payload_length);
client->write_buffer_len += payload_length;
if (!client->writable_event_source) {
client->writable_event_source = wl_event_loop_add_fd(
server.wl_event_loop, client->fd, WL_EVENT_WRITABLE,
ipc_client_handle_writable, client);
}
sway_log(SWAY_DEBUG, "Added IPC reply of type 0x%x to client %d queue: %s",
payload_type, client->fd, payload);
return true;
}
| {'content_hash': '20c27542041366fdb74fbe14e45cb399', 'timestamp': '', 'source': 'github', 'line_count': 961, 'max_line_length': 99, 'avg_line_length': 32.19146722164412, 'alnum_prop': 0.68470390483579, 'repo_name': '1ace/sway', 'id': '1bf5a05fe543b555734ac7e6e474dcfc5992e06a', 'size': '30936', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'sway/ipc-server.c', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'C', 'bytes': '1496495'}, {'name': 'Meson', 'bytes': '19914'}, {'name': 'Python', 'bytes': '5532'}, {'name': 'Roff', 'bytes': '2292'}, {'name': 'Shell', 'bytes': '10585'}]} |
/*
Package cmp defines a set of comparator interfaces and functions,
with default implementations for all standard comparable types.
Compare Results
---------------
comparing two values results in one of the following (int) constants:
cmp.LT = -1 // Less-Than
cmp.EQ = 0 // Equal
cmp.GT = 1 // Greater-Than
Type: cmp.T
-------------
Type cmp.T defines an interface that allows objects to export a comparator function:
type T interface {
Cmp(interface{}) int
}
Type: cmp.F
-------------
Type cmp.F defines a stand-alone comparator function:
type F func(a, b interface{}) int
Default Implementations
-----------------------
Default implementations are provided for all standard comparable types:
* byte
* float(32|64)
* int & int(8|16|32|64)
* rune
* string
* uint & uint(8|16|32|64)
Below are the definitions of the included int functions.
A complimentary set of functions exists for each of the
standard comparable types (listed above):
// cmp.Int is a cmp.T wrapper for basic type int.
// Example:
// var comparable cmp.T = cmp.Int(3)
type Int int
// cmp.Int::Cmp fulfills the cmp.T interface.
// b_ is expected to also be type cmp.Int.
func (a Int) Cmp(b_ interface{}) int
// cmp.F_Int is a cmp.F for type cmp.Int
// i.e. You can pass cmp.F_Int to functions
// requiring a cmp.F comparator
// a_ and b_ are expected to be type cmp.Int.
func F_Int(a_, b_ interface{}) int
// F_int is a cmp.F for basic type int
// i.e. You can pass cmp.F_int to functions
// requiring a cmp.F comparator
// a_ and b_ are expected to be basic type int.
func F_int(a_, b_ interface{}) int
// cmp.Compare_int is a convenience function
// for explicitly comparing basic type int.
func Compare_int(a, b int) int
Extras
------
The package also contains constructs for treating functions
and closures as cmp.T interfaces. See the file "cmp.go" for details.
License
-------
This package is released under the MIT license.
See the file "LICENSE" for more details.
Contributors
------------
David Farrell <[email protected]>
*/
package cmp
| {'content_hash': '1477b51e850bc26937d4e659b588d133', 'timestamp': '', 'source': 'github', 'line_count': 98, 'max_line_length': 84, 'avg_line_length': 21.448979591836736, 'alnum_prop': 0.6793529971455756, 'repo_name': 'iNamik/go_cmp', 'id': 'b913dee4c642fc515389d273212fe62f111ff416', 'size': '2102', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'doc.go', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Go', 'bytes': '19996'}]} |
<?php
//include the phal .phar file
include "[phalphar]";
| {'content_hash': '5258b84cdef9656c70208388dce15bad', 'timestamp': '', 'source': 'github', 'line_count': 4, 'max_line_length': 29, 'avg_line_length': 14.75, 'alnum_prop': 0.6779661016949152, 'repo_name': 'aparraga/phal', 'id': '645fa244c831443a0ca4fbf01ccdd12a4e5f4272', 'size': '59', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'app/index.php', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Batchfile', 'bytes': '1707'}, {'name': 'CSS', 'bytes': '163460'}, {'name': 'HTML', 'bytes': '11747373'}, {'name': 'Java', 'bytes': '410777'}, {'name': 'JavaScript', 'bytes': '147997'}, {'name': 'PHP', 'bytes': '6675966'}, {'name': 'PLpgSQL', 'bytes': '1325'}, {'name': 'Perl', 'bytes': '3594'}, {'name': 'Ruby', 'bytes': '578'}, {'name': 'SCSS', 'bytes': '16447'}, {'name': 'Shell', 'bytes': '5431'}, {'name': 'Smarty', 'bytes': '7974'}, {'name': 'XSLT', 'bytes': '106717'}, {'name': 'Yacc', 'bytes': '62595'}]} |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.