text
stringlengths 4
5.48M
| meta
stringlengths 14
6.54k
|
---|---|
{-# LANGUAGE CPP #-}
{-# LANGUAGE ConstraintKinds #-}
{-# LANGUAGE DataKinds #-}
{-# LANGUAGE FlexibleContexts #-}
{-# LANGUAGE FlexibleInstances #-}
{-# LANGUAGE ScopedTypeVariables #-}
{-# LANGUAGE TypeFamilies #-}
{-# LANGUAGE TypeOperators #-}
{-# LANGUAGE UndecidableInstances #-}
module Servant.Ruby.Internal where
import Servant.API
import Data.Proxy
import GHC.Exts (Constraint)
import GHC.TypeLits
data AjaxReq
class HasRB (layout :: *) where
type RB layout :: *
rubyFor :: Proxy layout -> AjaxReq -> RB layout
| {'content_hash': '04fe634b44648e221b1026a823d617c6', 'timestamp': '', 'source': 'github', 'line_count': 22, 'max_line_length': 51, 'avg_line_length': 26.727272727272727, 'alnum_prop': 0.6360544217687075, 'repo_name': 'parsonsmatt/servant-ruby', 'id': 'abcc508eb3facdd198c213260c073f31289f2906', 'size': '588', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/Servant/Ruby/Internal.hs', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Haskell', 'bytes': '1576'}]} |
from msrest.serialization import Model
from msrest.exceptions import HttpOperationError
class ErrorResponse(Model):
"""The error object.
:param error: Error.
:type error: ~azure.mgmt.resource.managementgroups.models.ErrorDetails
"""
_attribute_map = {
'error': {'key': 'error', 'type': 'ErrorDetails'},
}
def __init__(self, error=None):
super(ErrorResponse, self).__init__()
self.error = error
class ErrorResponseException(HttpOperationError):
"""Server responsed with exception of type: 'ErrorResponse'.
:param deserialize: A deserializer
:param response: Server response to be deserialized.
"""
def __init__(self, deserialize, response, *args):
super(ErrorResponseException, self).__init__(deserialize, response, 'ErrorResponse', *args)
| {'content_hash': '1cea87edd169f394f10b678c53ae0cc7', 'timestamp': '', 'source': 'github', 'line_count': 30, 'max_line_length': 99, 'avg_line_length': 27.633333333333333, 'alnum_prop': 0.6755126658624849, 'repo_name': 'AutorestCI/azure-sdk-for-python', 'id': 'd4af1d388d68ee2114db0e15d458065bc421a7b0', 'size': '1303', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'azure-mgmt-resource/azure/mgmt/resource/managementgroups/models/error_response.py', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Python', 'bytes': '34619070'}]} |
function matrix(n) {
const results = [];
for (let i = 0; i < n; i++) {
results.push([]);
}
let counter = 1;
let startColumn = 0;
let endColumn = n - 1;
let startRow = 0;
let endRow = n - 1;
while (startColumn <= endColumn && startRow <= endRow) {
// Top row
for (let i = startColumn; i <= endColumn; i++) {
results[startRow][i] = counter;
counter++;
}
startRow++;
// Right column
for (let i = startRow; i <= endRow; i++) {
results[i][endColumn] = counter;
counter++;
}
endColumn--;
// Bottom row
for (let i = endColumn; i >= startColumn; i--) {
results[endRow][i] = counter;
counter++;
}
endRow--;
// start column
for (let i = endRow; i >= startRow; i--) {
results[i][startColumn] = counter;
counter++;
}
startColumn++;
}
return results;
}
module.exports = matrix;
| {'content_hash': '6845853c2317bf025a109d0dc5d5a925', 'timestamp': '', 'source': 'github', 'line_count': 46, 'max_line_length': 58, 'avg_line_length': 19.782608695652176, 'alnum_prop': 0.5274725274725275, 'repo_name': 'spiresd55/code-playground', 'id': 'f1d0651e482f080e54421cf74e0c08079627ac1c', 'size': '1286', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'completed_exercises/matrix/index.js', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '899'}, {'name': 'HTML', 'bytes': '25468'}, {'name': 'JavaScript', 'bytes': '247128'}, {'name': 'TypeScript', 'bytes': '44268'}]} |
package com.wegas.app.jsf.controllers;
import com.wegas.core.ejb.GameFacade;
import com.wegas.core.ejb.PlayerFacade;
import com.wegas.core.exception.internal.WegasNoResultException;
import com.wegas.core.persistence.game.Game;
import com.wegas.core.persistence.game.GameModel;
import java.io.IOException;
import java.io.Serializable;
import javax.annotation.PostConstruct;
import javax.ejb.EJB;
import javax.enterprise.context.RequestScoped;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.ManagedProperty;
import javax.faces.context.ExternalContext;
import javax.faces.context.FacesContext;
import javax.inject.Inject;
/**
*
* @author Francois-Xavier Aeberhard (fx at red-agent.com)
*/
@ManagedBean(name = "singleLobbyController")
@RequestScoped
public class SingleLobbyController implements Serializable {
/**
*
*/
@ManagedProperty("#{param.token}")
private String token;
/**
*
*/
@EJB
private GameFacade gameFacade;
/**
*
*/
@EJB
private PlayerFacade playerFacade;
/**
*
*/
@Inject
ErrorController errorController;
/**
*
*/
private Game currentGame = null;
/**
*
* @fixme rights management
*
*/
@PostConstruct
public void init() {
final ExternalContext externalContext = FacesContext.getCurrentInstance().getExternalContext();
if (token != null) {
currentGame = gameFacade.findByToken(token);
if (currentGame != null) { // 1st case: token is associated with a game
try {
playerFacade.findCurrentPlayer(currentGame);
try {
externalContext.dispatch("game-play.xhtml?gameId=" + currentGame.getId());// display game page
} catch (IOException ex) {
}
} catch (WegasNoResultException e) {
// Nothing to do. stay on current page so player will choose his team
}
//} else { // 2nd case: token is associated with a team
// final Team currentTeam = teamFacade.findByToken(token);
// if (currentTeam != null) {
// try {
// playerFacade.findCurrentPlayer(currentTeam.getGame());
// } catch (NoResultException etp) { // Player has not joined yet
// if (SecurityHelper.isAnyPermitted(currentTeam.getGame(), Arrays.asList("Token", "TeamToken", "View"))) {
// teamFacade.joinTeam(currentTeam, userFacade.getCurrentUser()); // so we join him
// } else {
// externalContext.dispatch("/wegas-app/view/error/accessdenied.xhtml"); // not allowed
// }
// }
// externalContext.dispatch("game-play.xhtml?gameId=" + currentTeam.getGame().getId());// display game page
// } else {
// externalContext.dispatch("/wegas-app/view/error/accessdenied.xhtml"); // no game
// }
//}
} else {
errorController.dispatch("The game you are looking for could not be found.");
}
} else {
errorController.dispatch("The game you are looking for could not be found.");
}
}
/**
* @return the token
*/
public String getToken() {
return token;
}
/**
* @param token the token to set
*/
public void setToken(String token) {
this.token = token;
}
/**
* @return the currentGame
*/
public Game getCurrentGame() {
return currentGame;
}
/**
* @param currentGame the currentGame to set
*/
public void setCurrentGame(Game currentGame) {
this.currentGame = currentGame;
}
/**
* @return the current game model
*/
public GameModel getCurrentGameModel() {
return currentGame.getGameModel();
}
}
| {'content_hash': '92e1ec893a8350c0391127422f011016', 'timestamp': '', 'source': 'github', 'line_count': 134, 'max_line_length': 134, 'avg_line_length': 31.71641791044776, 'alnum_prop': 0.5510588235294117, 'repo_name': 'ghiringh/Wegas', 'id': '2bfa7b5f89e99f28fe0b6e976de2f7ef822ed52f', 'size': '4410', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'wegas-app/src/main/java/com/wegas/app/jsf/controllers/SingleLobbyController.java', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '903473'}, {'name': 'HTML', 'bytes': '195241'}, {'name': 'Java', 'bytes': '1757507'}, {'name': 'JavaScript', 'bytes': '4366779'}]} |
from datetime import timedelta
from django.core.management import call_command
from tests.test_case import AppTestCase
from wagtailstreamforms.models import Form, FormSubmission
class Tests(AppTestCase):
fixtures = ["test"]
def test_command(self):
form = Form.objects.get(pk=1)
to_keep = FormSubmission.objects.create(form=form, form_data={})
to_delete = FormSubmission.objects.create(form=form, form_data={})
to_delete.submit_time = to_delete.submit_time - timedelta(days=2)
to_delete.save()
call_command("prunesubmissions", 1)
FormSubmission.objects.get(pk=to_keep.pk)
with self.assertRaises(FormSubmission.DoesNotExist):
FormSubmission.objects.get(pk=to_delete.pk)
| {'content_hash': '4cca3b3599e9153138fb9bf4ae4582db', 'timestamp': '', 'source': 'github', 'line_count': 24, 'max_line_length': 74, 'avg_line_length': 31.666666666666668, 'alnum_prop': 0.7, 'repo_name': 'AccentDesign/wagtailstreamforms', 'id': '71bcb5296a3739d9e37fc034c60c318a915da6a2', 'size': '760', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'tests/management/test_prunesubmissions.py', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Dockerfile', 'bytes': '690'}, {'name': 'HTML', 'bytes': '14735'}, {'name': 'JavaScript', 'bytes': '213'}, {'name': 'Makefile', 'bytes': '438'}, {'name': 'Python', 'bytes': '189375'}, {'name': 'SCSS', 'bytes': '2257'}, {'name': 'Shell', 'bytes': '559'}]} |
package proc
import (
"errors"
"fmt"
"math"
"strings"
"github.com/go-delve/delve/pkg/dwarf/op"
)
// Registers is an interface for a generic register type. The
// interface encapsulates the generic values / actions
// we need independent of arch. The concrete register types
// will be different depending on OS/Arch.
type Registers interface {
PC() uint64
SP() uint64
BP() uint64
LR() uint64
TLS() uint64
// GAddr returns the address of the G variable if it is known, 0 and false otherwise
GAddr() (uint64, bool)
Slice(floatingPoint bool) ([]Register, error)
// Copy returns a copy of the registers that is guaranteed not to change
// when the registers of the associated thread change.
Copy() (Registers, error)
}
// Register represents a CPU register.
type Register struct {
Name string
Reg *op.DwarfRegister
}
// AppendUint64Register will create a new Register struct with the name and value
// specified and append it to the `regs` slice.
func AppendUint64Register(regs []Register, name string, value uint64) []Register {
return append(regs, Register{name, op.DwarfRegisterFromUint64(value)})
}
// AppendBytesRegister will create a new Register struct with the name and value
// specified and append it to the `regs` slice.
func AppendBytesRegister(regs []Register, name string, value []byte) []Register {
return append(regs, Register{name, op.DwarfRegisterFromBytes(value)})
}
// ErrUnknownRegister is returned when the value of an unknown
// register is requested.
var ErrUnknownRegister = errors.New("unknown register")
type flagRegisterDescr []flagDescr
type flagDescr struct {
name string
mask uint64
}
var mxcsrDescription flagRegisterDescr = []flagDescr{
{"FZ", 1 << 15},
{"RZ/RN", 1<<14 | 1<<13},
{"PM", 1 << 12},
{"UM", 1 << 11},
{"OM", 1 << 10},
{"ZM", 1 << 9},
{"DM", 1 << 8},
{"IM", 1 << 7},
{"DAZ", 1 << 6},
{"PE", 1 << 5},
{"UE", 1 << 4},
{"OE", 1 << 3},
{"ZE", 1 << 2},
{"DE", 1 << 1},
{"IE", 1 << 0},
}
var eflagsDescription flagRegisterDescr = []flagDescr{
{"CF", 1 << 0},
{"", 1 << 1},
{"PF", 1 << 2},
{"AF", 1 << 4},
{"ZF", 1 << 6},
{"SF", 1 << 7},
{"TF", 1 << 8},
{"IF", 1 << 9},
{"DF", 1 << 10},
{"OF", 1 << 11},
{"IOPL", 1<<12 | 1<<13},
{"NT", 1 << 14},
{"RF", 1 << 16},
{"VM", 1 << 17},
{"AC", 1 << 18},
{"VIF", 1 << 19},
{"VIP", 1 << 20},
{"ID", 1 << 21},
}
func (descr flagRegisterDescr) Mask() uint64 {
var r uint64
for _, f := range descr {
r = r | f.mask
}
return r
}
func (descr flagRegisterDescr) Describe(reg uint64, bitsize int) string {
var r []string
for _, f := range descr {
if f.name == "" {
continue
}
// rbm is f.mask with only the right-most bit set:
// 0001 1100 -> 0000 0100
rbm := f.mask & -f.mask
if rbm == f.mask {
if reg&f.mask != 0 {
r = append(r, f.name)
}
} else {
x := (reg & f.mask) >> uint64(math.Log2(float64(rbm)))
r = append(r, fmt.Sprintf("%s=%x", f.name, x))
}
}
if reg & ^descr.Mask() != 0 {
r = append(r, fmt.Sprintf("unknown_flags=%x", reg&^descr.Mask()))
}
return fmt.Sprintf("%#0*x\t[%s]", bitsize/4, reg, strings.Join(r, " "))
}
| {'content_hash': '5fac7ad48d7df82fdd182c2e35a6f8f2', 'timestamp': '', 'source': 'github', 'line_count': 127, 'max_line_length': 85, 'avg_line_length': 24.511811023622048, 'alnum_prop': 0.6174108576935432, 'repo_name': 'go-delve/delve', 'id': '185d0615c5eb8fd881fef343a12ddc512255e5c1', 'size': '3113', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'pkg/proc/registers.go', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Assembly', 'bytes': '3179'}, {'name': 'C', 'bytes': '2657334'}, {'name': 'Go', 'bytes': '2792924'}, {'name': 'Kotlin', 'bytes': '7688'}, {'name': 'Makefile', 'bytes': '970'}, {'name': 'PowerShell', 'bytes': '3085'}, {'name': 'Python', 'bytes': '599'}, {'name': 'Shell', 'bytes': '5109'}, {'name': 'Starlark', 'bytes': '2024'}]} |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>riscv: Not compatible 👼</title>
<link rel="shortcut icon" type="image/png" href="../../../../../favicon.png" />
<link href="../../../../../bootstrap.min.css" rel="stylesheet">
<link href="../../../../../bootstrap-custom.css" rel="stylesheet">
<link href="//maxcdn.bootstrapcdn.com/font-awesome/4.2.0/css/font-awesome.min.css" rel="stylesheet">
<script src="../../../../../moment.min.js"></script>
<!-- HTML5 Shim and Respond.js IE8 support of HTML5 elements and media queries -->
<!-- WARNING: Respond.js doesn't work if you view the page via file:// -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/html5shiv/3.7.2/html5shiv.min.js"></script>
<script src="https://oss.maxcdn.com/respond/1.4.2/respond.min.js"></script>
<![endif]-->
</head>
<body>
<div class="container">
<div class="navbar navbar-default" role="navigation">
<div class="container-fluid">
<div class="navbar-header">
<a class="navbar-brand" href="../../../../.."><i class="fa fa-lg fa-flag-checkered"></i> Coq bench</a>
</div>
<div id="navbar" class="collapse navbar-collapse">
<ul class="nav navbar-nav">
<li><a href="../..">clean / released</a></li>
<li class="active"><a href="">8.7.1+1 / riscv - 0.0.3</a></li>
</ul>
</div>
</div>
</div>
<div class="article">
<div class="row">
<div class="col-md-12">
<a href="../..">« Up</a>
<h1>
riscv
<small>
0.0.3
<span class="label label-info">Not compatible 👼</span>
</small>
</h1>
<p>📅 <em><script>document.write(moment("2022-11-10 23:24:19 +0000", "YYYY-MM-DD HH:mm:ss Z").fromNow());</script> (2022-11-10 23:24:19 UTC)</em><p>
<h2>Context</h2>
<pre># Packages matching: installed
# Name # Installed # Synopsis
base-bigarray base
base-num base Num library distributed with the OCaml compiler
base-ocamlbuild base OCamlbuild binary and libraries distributed with the OCaml compiler
base-threads base
base-unix base
camlp5 7.14 Preprocessor-pretty-printer of OCaml
conf-findutils 1 Virtual package relying on findutils
conf-perl 2 Virtual package relying on perl
coq 8.7.1+1 Formal proof management system
num 0 The Num library for arbitrary-precision integer and rational arithmetic
ocaml 4.02.3 The OCaml compiler (virtual package)
ocaml-base-compiler 4.02.3 Official 4.02.3 release
ocaml-config 1 OCaml Switch Configuration
ocamlfind 1.9.5 A library manager for OCaml
# opam file:
opam-version: "2.0"
authors: [
"Massachusetts Institute of Technology"
]
maintainer: "Jason Gross <[email protected]>"
homepage: "https://github.com/mit-plv/riscv-coq"
bug-reports: "https://github.com/mit-plv/riscv-coq/issues"
license: "BSD-3-Clause"
build: [
[make "-j%{jobs}%" "EXTERNAL_DEPENDENCIES=1" "all"]
]
install: [make "EXTERNAL_DEPENDENCIES=1" "install"]
depends: [
"coq" {>= "8.15~"}
"coq-coqutil" {= "0.0.2"}
"coq-record-update" {>= "0.3.0"}
]
dev-repo: "git+https://github.com/mit-plv/riscv-coq.git"
synopsis: "RISC-V Specification in Coq, somewhat experimental"
tags: ["logpath:riscv"]
url {
src: "https://github.com/mit-plv/riscv-coq/archive/refs/tags/v0.0.3.tar.gz"
checksum: "sha512=55c6a2aa84c89b5b4224729ccad23504d906d174d8bab9b5e1ff62dd7e76efef4935978c3ba517870d25700a1e563e2b352bb3fba94936807561840f26af75e8"
}
</pre>
<h2>Lint</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Dry install 🏜️</h2>
<p>Dry install with the current Coq version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam install -y --show-action coq-riscv.0.0.3 coq.8.7.1+1</code></dd>
<dt>Return code</dt>
<dd>5120</dd>
<dt>Output</dt>
<dd><pre>[NOTE] Package coq is already installed (current version is 8.7.1+1).
The following dependencies couldn't be met:
- coq-riscv -> coq >= 8.15~ -> ocaml >= 4.05.0
base of this switch (use `--unlock-base' to force)
No solution found, exiting
</pre></dd>
</dl>
<p>Dry install without Coq/switch base, to test if the problem was incompatibility with the current Coq/OCaml version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam remove -y coq; opam install -y --show-action --unlock-base coq-riscv.0.0.3</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Install dependencies</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>0 s</dd>
</dl>
<h2>Install 🚀</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>0 s</dd>
</dl>
<h2>Installation size</h2>
<p>No files were installed.</p>
<h2>Uninstall 🧹</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Missing removes</dt>
<dd>
none
</dd>
<dt>Wrong removes</dt>
<dd>
none
</dd>
</dl>
</div>
</div>
</div>
<hr/>
<div class="footer">
<p class="text-center">
Sources are on <a href="https://github.com/coq-bench">GitHub</a> © Guillaume Claret 🐣
</p>
</div>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
<script src="../../../../../bootstrap.min.js"></script>
</body>
</html>
| {'content_hash': '500a50feebf1f5c4e792362c3876765f', 'timestamp': '', 'source': 'github', 'line_count': 166, 'max_line_length': 159, 'avg_line_length': 41.566265060240966, 'alnum_prop': 0.5395652173913044, 'repo_name': 'coq-bench/coq-bench.github.io', 'id': 'a2d72cb16b363e6581ce4064c8dfafbe665c61e2', 'size': '6925', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'clean/Linux-x86_64-4.02.3-2.0.6/released/8.7.1+1/riscv/0.0.3.html', 'mode': '33188', 'license': 'mit', 'language': []} |
<!doctype html>
<html>
<title>npm-stop</title>
<meta http-equiv="content-type" value="text/html;utf-8">
<link rel="stylesheet" type="text/css" href="../../static/style.css">
<link rel="canonical" href="https://www.npmjs.org/doc/cli/npm-stop.html">
<script async=true src="../../static/toc.js"></script>
<body>
<div id="wrapper">
<h1><a href="../cli/npm-stop.html">npm-stop</a></h1> <p>Stop a package</p>
<h2 id="synopsis">SYNOPSIS</h2>
<pre><code>npm stop [-- <args>]
</code></pre><h2 id="description">DESCRIPTION</h2>
<p>This runs a package's "stop" script, if one was provided.</p>
<h2 id="see-also">SEE ALSO</h2>
<ul>
<li><a href="../cli/npm-run-script.html">npm-run-script(1)</a></li>
<li><a href="../misc/npm-scripts.html">npm-scripts(7)</a></li>
<li><a href="../cli/npm-test.html">npm-test(1)</a></li>
<li><a href="../cli/npm-start.html">npm-start(1)</a></li>
<li><a href="../cli/npm-restart.html">npm-restart(1)</a></li>
</ul>
</div>
<table border=0 cellspacing=0 cellpadding=0 id=npmlogo>
<tr><td style="width:180px;height:10px;background:rgb(237,127,127)" colspan=18> </td></tr>
<tr><td rowspan=4 style="width:10px;height:10px;background:rgb(237,127,127)"> </td><td style="width:40px;height:10px;background:#fff" colspan=4> </td><td style="width:10px;height:10px;background:rgb(237,127,127)" rowspan=4> </td><td style="width:40px;height:10px;background:#fff" colspan=4> </td><td rowspan=4 style="width:10px;height:10px;background:rgb(237,127,127)"> </td><td colspan=6 style="width:60px;height:10px;background:#fff"> </td><td style="width:10px;height:10px;background:rgb(237,127,127)" rowspan=4> </td></tr>
<tr><td colspan=2 style="width:20px;height:30px;background:#fff" rowspan=3> </td><td style="width:10px;height:10px;background:rgb(237,127,127)" rowspan=3> </td><td style="width:10px;height:10px;background:#fff" rowspan=3> </td><td style="width:20px;height:10px;background:#fff" rowspan=4 colspan=2> </td><td style="width:10px;height:20px;background:rgb(237,127,127)" rowspan=2> </td><td style="width:10px;height:10px;background:#fff" rowspan=3> </td><td style="width:20px;height:10px;background:#fff" rowspan=3 colspan=2> </td><td style="width:10px;height:10px;background:rgb(237,127,127)" rowspan=3> </td><td style="width:10px;height:10px;background:#fff" rowspan=3> </td><td style="width:10px;height:10px;background:rgb(237,127,127)" rowspan=3> </td></tr>
<tr><td style="width:10px;height:10px;background:#fff" rowspan=2> </td></tr>
<tr><td style="width:10px;height:10px;background:#fff"> </td></tr>
<tr><td style="width:60px;height:10px;background:rgb(237,127,127)" colspan=6> </td><td colspan=10 style="width:10px;height:10px;background:rgb(237,127,127)"> </td></tr>
<tr><td colspan=5 style="width:50px;height:10px;background:#fff"> </td><td style="width:40px;height:10px;background:rgb(237,127,127)" colspan=4> </td><td style="width:90px;height:10px;background:#fff" colspan=9> </td></tr>
</table>
<p id="footer">npm-stop — [email protected]</p>
| {'content_hash': '386a7305980a40b5670a511695fa9160', 'timestamp': '', 'source': 'github', 'line_count': 38, 'max_line_length': 807, 'avg_line_length': 82.39473684210526, 'alnum_prop': 0.6940274672628554, 'repo_name': 'Tearund/stories', 'id': '2047d8e85417b2232688c109156deec3acb98823', 'size': '3131', 'binary': False, 'copies': '3', 'ref': 'refs/heads/master', 'path': 'node_modules/cordova/node_modules/cordova-lib/node_modules/npm/html/doc/cli/npm-stop.html', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Batchfile', 'bytes': '4027'}, {'name': 'C', 'bytes': '1025'}, {'name': 'CSS', 'bytes': '1028'}, {'name': 'HTML', 'bytes': '4766'}, {'name': 'Java', 'bytes': '6311'}, {'name': 'JavaScript', 'bytes': '24488'}, {'name': 'Objective-C', 'bytes': '221904'}, {'name': 'Shell', 'bytes': '2480'}]} |
"""Implement operations for branch-based reviews."""
# =============================================================================
# CONTENTS
# -----------------------------------------------------------------------------
# abdt_branch
#
# Public Classes:
# Branch
# .is_abandoned
# .is_null
# .is_new
# .is_status_bad_pre_review
# .is_status_bad_land
# .is_status_bad_abandoned
# .is_status_bad
# .has_new_commits
# .base_branch_name
# .review_branch_hash
# .review_branch_name
# .review_id_or_none
# .get_author_names_emails
# .get_any_author_emails
# .get_repo_name
# .get_browse_url
# .describe
# .describe_new_commits
# .make_message_digest
# .make_raw_diff
# .verify_review_branch_base
# .get_commit_message_from_tip
# .abandon
# .remove
# .clear_mark
# .mark_bad_land
# .mark_bad_abandoned
# .mark_bad_in_review
# .mark_new_bad_in_review
# .mark_bad_pre_review
# .mark_ok_in_review
# .mark_ok_new_review
# .land
#
# Public Functions:
# calc_is_ok
#
# -----------------------------------------------------------------------------
# (this contents block is generated, edits will be lost)
# =============================================================================
# TODO: write test driver
from __future__ import absolute_import
import phlgit_checkout
import phlgit_log
import phlgit_push
import phlgit_revparse
import phlgitu_ref
import phlsys_textconvert
import abdt_differ
import abdt_errident
import abdt_exception
import abdt_lander
import abdt_naming
import abdt_tryloop
# TODO: allow this to be passed in
_MAX_DIFF_SIZE = int(1.5 * 1024 * 1024)
def calc_is_ok(branch):
"""Return True if the supplied 'branch' is ok, False if bad, else None.
Note that a branch can be 'null' in which case we return None.
:branch: the Branch to examine
:returns: bool status of the branch
"""
assert branch is not None
if branch.is_null() or branch.is_new() or branch.is_abandoned():
return None
return not branch.is_status_bad()
class Branch(object):
def __init__(
self,
repo,
review_branch,
review_hash,
tracking_branch,
tracking_hash,
lander,
repo_name,
browse_url=None):
"""Create a new relationship tracker for the supplied branch names.
:repo: a callable supporting git commands, e.g. repo("status")
:review_branch: the abdt_gittypes.GitReviewBranch
:review_hash: the commit hash of the branch or None
:tracking_branch: the abdt_gittypes.GitWorkingBranch
:tracking_hash: the commit hash of the branch or None
:lander: a lander conformant to abdt_lander
:repo_name: a short string to identify the repo to humans
:browse_url: a URL to browse the branch or repo (may be None)
"""
self._repo = repo
self._review_branch = review_branch
self._review_hash = review_hash
self._tracking_branch = tracking_branch
self._tracking_hash = tracking_hash
self._lander = lander
assert self._review_branch_valid_or_none()
assert self._tracking_branch_valid_or_none()
self._repo_name = repo_name
self._browse_url = browse_url
assert self._repo_name is not None
def _review_branch_valid_or_none(self):
if not self._has_review_branch():
return True
else:
return isinstance(
self._review_branch,
abdt_naming.ReviewBranch)
def _tracking_branch_valid_or_none(self):
if not self._has_tracking_branch():
return True
else:
return isinstance(
self._tracking_branch,
abdt_naming.TrackerBranch)
def _has_review_branch(self):
return self._review_branch is not None
def _has_tracking_branch(self):
return self._tracking_branch is not None
def is_abandoned(self):
"""Return True if the author's branch no longer exists."""
return not self._has_review_branch() and self._has_tracking_branch()
def is_null(self):
"""Return True if we don't have any data."""
no_review_branch = not self._has_review_branch()
no_tracking_branch = not self._has_tracking_branch()
return no_review_branch and no_tracking_branch
def is_new(self):
"""Return True if we haven't marked the author's branch."""
return self._has_review_branch() and not self._has_tracking_branch()
def is_status_bad_pre_review(self):
"""Return True if the author's branch is marked 'bad pre-review'."""
if self._has_tracking_branch():
return abdt_naming.isStatusBadPreReview(self._tracking_branch)
else:
return False
def is_status_bad_land(self):
"""Return True if the author's branch is marked 'bad land'."""
if self._has_tracking_branch():
return abdt_naming.isStatusBadLand(self._tracking_branch)
else:
return False
def is_status_bad_abandoned(self):
"""Return True if the author's branch is marked 'bad abandoned'."""
if self._has_tracking_branch():
branch = self._tracking_branch
return branch.status == abdt_naming.WB_STATUS_BAD_ABANDONED
else:
return False
def is_status_bad(self):
"""Return True if the author's branch is marked any bad status."""
if self._has_tracking_branch():
return abdt_naming.isStatusBad(self._tracking_branch)
else:
return False
def has_new_commits(self):
"""Return True if the author's branch is different since marked."""
if self.is_new():
return True
else:
return self._review_hash != self._tracking_hash
def base_branch_name(self):
"""Return the string name of the branch the review will land on."""
if self._review_branch:
return self._review_branch.base
return self._tracking_branch.base
def review_branch_hash(self):
"""Return the string hash of the review branch or None."""
return self._review_hash
def review_branch_name(self):
"""Return the string name of the branch the review is based on."""
if self._review_branch:
return self._review_branch.branch
return self._tracking_branch.review_name
def review_id_or_none(self):
"""Return the int id of the review or 'None' if there isn't one."""
if not self._tracking_branch:
return None
review_id = None
try:
review_id = int(self._tracking_branch.id)
except ValueError:
pass
return review_id
def get_author_names_emails(self):
"""Return a list of (name, email) tuples from the branch."""
hashes = self._get_commit_hashes()
# names and emails are only mentioned once, in the order that they
# appear. reverse the order so that the the most recent commit is
# considered first.
hashes.reverse()
names_emails = phlgit_log.get_author_names_emails_from_hashes(
self._repo, hashes)
names_emails.reverse()
return names_emails
def get_any_author_emails(self):
"""Return a list of emails from the branch.
If the branch has an invalid base or has no history against the base
then resort to using the whole history.
Useful if 'get_author_names_emails' fails.
"""
if phlgit_revparse.get_sha1_or_none(
self._repo, self._review_branch.remote_base) is None:
hashes = phlgit_log.get_last_n_commit_hashes_from_ref(
self._repo, 1, self._review_branch.remote_branch)
else:
hashes = self._get_commit_hashes()
if not hashes:
hashes = phlgit_log.get_last_n_commit_hashes_from_ref(
self._repo, 1, self._review_branch.remote_branch)
committers = phlgit_log.get_author_names_emails_from_hashes(
self._repo, hashes)
emails = [committer[1] for committer in committers]
return emails
def get_repo_name(self):
"""Return the human name for the repo the branch came from."""
return self._repo_name
def get_browse_url(self):
"""Return the url to browse this branch, may be None."""
return self._browse_url
def _get_commit_hashes(self):
hashes = self._repo.get_range_hashes(
self._review_branch.remote_base,
self._review_branch.remote_branch)
return hashes
def describe(self):
"""Return a string description of this branch for a human to read."""
branch_description = "(null branch)"
if not self.is_null():
branch_description = self.review_branch_name()
if self.is_abandoned():
branch_description += " (abandoned)"
return "{}, {}".format(self.get_repo_name(), branch_description)
def describe_new_commits(self):
"""Return a string description of the new commits on the branch."""
hashes = None
previous = None
latest = self._review_branch.remote_branch
if self.is_new():
previous = self._review_branch.remote_base
else:
previous = self._tracking_branch.remote_branch
hashes = self._repo.get_range_hashes(previous, latest)
hashes.reverse()
revisions = self._repo.make_revisions_from_hashes(hashes)
message = ""
for r in revisions:
message += r.abbrev_hash + " " + r.subject + "\n"
return phlsys_textconvert.ensure_ascii(message)
def make_message_digest(self):
"""Return a string digest of the commit messages on the branch.
The digest is comprised of the title from the earliest commit
unique to the branch and all of the message bodies from the
unique commits on the branch.
"""
hashes = self._get_commit_hashes()
revisions = self._repo.make_revisions_from_hashes(hashes)
message = revisions[0].subject + "\n\n"
for r in revisions:
message += r.message
return phlsys_textconvert.ensure_ascii(message)
def make_raw_diff(self):
"""Return an abdt_differ.DiffResult of the changes on the branch.
If the diff would exceed the pre-specified max diff size then take
measures to reduce the diff.
"""
# checkout the 'to' branch, otherwise we won't take into account any
# changes to .gitattributes files
phlgit_checkout.branch(self._repo, self._review_branch.remote_branch)
try:
return abdt_differ.make_raw_diff(
self._repo,
self._review_branch.remote_base,
self._review_branch.remote_branch,
_MAX_DIFF_SIZE)
except abdt_differ.NoDiffError:
raise abdt_exception.NoDiffException(
self.base_branch_name(),
self.review_branch_name(),
self.review_branch_hash())
def _is_based_on(self, name, base):
# TODO: actually do this
return True
def verify_review_branch_base(self):
"""Raise exception if review branch has invalid base."""
if self._review_branch.base not in self._repo.get_remote_branches():
raise abdt_exception.MissingBaseException(
self._review_branch.branch,
self._review_branch.description,
self._review_branch.base)
if not self._is_based_on(
self._review_branch.branch, self._review_branch.base):
raise abdt_exception.AbdUserException(
"'" + self._review_branch.branch +
"' is not based on '" + self._review_branch.base + "'")
def get_commit_message_from_tip(self):
"""Return string commit message from latest commit on branch."""
hashes = self._get_commit_hashes()
revision = phlgit_log.make_revision_from_hash(self._repo, hashes[-1])
message = revision.subject + "\n"
message += "\n"
message += revision.message + "\n"
return phlsys_textconvert.ensure_ascii(message)
def _push_delete_review_branch(self):
def action():
self._repo.push_delete(self._review_branch.branch)
self._tryloop(action, abdt_errident.PUSH_DELETE_REVIEW)
def _push_delete_tracking_branch(self):
def action():
self._repo.push_delete(self._tracking_branch.branch)
self._tryloop(action, abdt_errident.PUSH_DELETE_TRACKING)
def abandon(self):
"""Remove information associated with the abandoned review branch."""
# TODO: raise if the branch is not actually abandoned by the user
self._push_delete_tracking_branch()
self._tracking_branch = None
self._tracking_hash = None
def remove(self):
"""Remove review branch and tracking branch."""
self._repo.archive_to_abandoned(
self._review_hash,
self.review_branch_name(),
self._tracking_branch.base)
# push the abandoned archive, don't escalate if it fails to push
try:
# XXX: oddly pylint complains if we call push_landed() directly:
# "Using method (_tryloop) as an attribute (not invoked)"
def push_abandoned():
self._repo.push_abandoned()
self._tryloop(
push_abandoned,
abdt_errident.PUSH_ABANDONED_ARCHIVE)
except Exception:
# XXX: don't worry if we can't push the landed, this is most
# likely a permissioning issue but not a showstopper.
# we should probably nag on the review instead.
pass
self._push_delete_review_branch()
self._push_delete_tracking_branch()
self._review_branch = None
self._review_hash = None
self._tracking_branch = None
self._tracking_hash = None
def clear_mark(self):
"""Clear status and last commit associated with the review branch."""
self._push_delete_tracking_branch()
self._tracking_branch = None
self._tracking_hash = None
def mark_bad_land(self):
"""Mark the current version of the review branch as 'bad land'."""
assert self.review_id_or_none() is not None
self._tryloop(
lambda: self._push_status(abdt_naming.WB_STATUS_BAD_LAND),
abdt_errident.MARK_BAD_LAND)
def mark_bad_abandoned(self):
"""Mark the current version of the review branch as 'bad abandoned'."""
assert self.review_id_or_none() is not None
self._tryloop(
lambda: self._push_status(abdt_naming.WB_STATUS_BAD_ABANDONED),
abdt_errident.MARK_BAD_ABANDONED)
def mark_bad_in_review(self):
"""Mark the current version of the review branch as 'bad in review'."""
assert self.review_id_or_none() is not None
self._tryloop(
lambda: self._push_status(abdt_naming.WB_STATUS_BAD_INREVIEW),
abdt_errident.MARK_BAD_IN_REVIEW)
def mark_new_bad_in_review(self, revision_id):
"""Mark the current version of the review branch as 'bad in review'."""
assert self.review_id_or_none() is None
def action():
if not self.is_new():
# 'push_bad_new_in_review' wont clean up our existing tracker
self._push_delete_tracking_branch()
self._push_new(
abdt_naming.WB_STATUS_BAD_INREVIEW,
revision_id)
self._tryloop(action, abdt_errident.MARK_NEW_BAD_IN_REVIEW)
def mark_bad_pre_review(self):
"""Mark this version of the review branch as 'bad pre review'."""
assert self.review_id_or_none() is None
assert self.is_status_bad_pre_review() or self.is_new()
# early out if this operation is redundant, pushing is expensive
if self.is_status_bad_pre_review() and not self.has_new_commits():
return
def action():
self._push_new(
abdt_naming.WB_STATUS_BAD_PREREVIEW,
None)
self._tryloop(
action, abdt_errident.MARK_BAD_PRE_REVIEW)
def mark_ok_in_review(self):
"""Mark this version of the review branch as 'ok in review'."""
assert self.review_id_or_none() is not None
self._tryloop(
lambda: self._push_status(abdt_naming.WB_STATUS_OK),
abdt_errident.MARK_OK_IN_REVIEW)
def mark_ok_new_review(self, revision_id):
"""Mark this version of the review branch as 'ok in review'."""
assert self.review_id_or_none() is None
def action():
if not self.is_new():
# 'push_bad_new_in_review' wont clean up our existing tracker
self._push_delete_tracking_branch()
self._push_new(
abdt_naming.WB_STATUS_OK,
revision_id)
self._tryloop(action, abdt_errident.MARK_OK_NEW_REVIEW)
def land(self, author_name, author_email, message):
"""Integrate the branch into the base and remove the review branch."""
self._repo.checkout_forced_new_branch(
self._tracking_branch.base,
self._tracking_branch.remote_base)
try:
result = self._lander(
self._repo,
self._tracking_branch.remote_branch,
author_name,
author_email,
message)
except abdt_lander.LanderException as e:
self._repo("reset", "--hard") # fix the working copy
raise abdt_exception.LandingException(
str(e),
self.review_branch_name(),
self._tracking_branch.base)
landing_hash = phlgit_revparse.get_sha1(
self._repo, self._tracking_branch.base)
# don't tryloop here as it's more expected that we can't push the base
# due to permissioning or some other error
try:
self._repo.push(self._tracking_branch.base)
except Exception as e:
raise abdt_exception.LandingPushBaseException(
str(e),
self.review_branch_name(),
self._tracking_branch.base)
self._tryloop(
lambda: self._repo.push_delete(
self._tracking_branch.branch,
self.review_branch_name()),
abdt_errident.PUSH_DELETE_LANDED)
self._repo.archive_to_landed(
self._tracking_hash,
self.review_branch_name(),
self._tracking_branch.base,
landing_hash,
message)
# push the landing archive, don't escalate if it fails to push
try:
# XXX: oddly pylint complains if we call push_landed() directly:
# "Using method (_tryloop) as an attribute (not invoked)"
def push_landed():
self._repo.push_landed()
self._tryloop(
push_landed,
abdt_errident.PUSH_LANDING_ARCHIVE)
except Exception:
# XXX: don't worry if we can't push the landed, this is most
# likely a permissioning issue but not a showstopper.
# we should probably nag on the review instead.
pass
self._review_branch = None
self._review_hash = None
self._tracking_branch = None
self._tracking_hash = None
return result
def _push_status(self, status):
old_branch = self._tracking_branch.branch
self._tracking_branch.update_status(status)
new_branch = self._tracking_branch.branch
if old_branch == new_branch:
phlgit_push.push_asymmetrical_force(
self._repo,
self._review_branch.remote_branch,
phlgitu_ref.make_local(new_branch),
self._tracking_branch.remote)
else:
phlgit_push.move_asymmetrical(
self._repo,
self._review_branch.remote_branch,
phlgitu_ref.make_local(old_branch),
phlgitu_ref.make_local(new_branch),
self._repo.get_remote())
self._tracking_hash = self._review_hash
def _push_new(self, status, revision_id):
tracking_branch = self._review_branch.make_tracker(
status, revision_id)
phlgit_push.push_asymmetrical_force(
self._repo,
self._review_branch.remote_branch,
phlgitu_ref.make_local(tracking_branch.branch),
tracking_branch.remote)
self._tracking_branch = tracking_branch
self._tracking_hash = self._review_hash
def _tryloop(self, f, identifier):
return abdt_tryloop.tryloop(f, identifier, self.describe())
# -----------------------------------------------------------------------------
# Copyright (C) 2013-2014 Bloomberg Finance L.P.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# ------------------------------ END-OF-FILE ----------------------------------
| {'content_hash': '7e19f938becb8eca646b05639a647d32', 'timestamp': '', 'source': 'github', 'line_count': 623, 'max_line_length': 79, 'avg_line_length': 35.258426966292134, 'alnum_prop': 0.5866794136392607, 'repo_name': 'valhallasw/phabricator-tools', 'id': '14468f155655f4eca7646f095836170115a5608a', 'size': '21966', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'py/abd/abdt_branch.py', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'C++', 'bytes': '342'}, {'name': 'Puppet', 'bytes': '4246'}, {'name': 'Python', 'bytes': '964066'}, {'name': 'Ruby', 'bytes': '2000'}, {'name': 'Shell', 'bytes': '128202'}]} |
package com.eyeem.recyclerviewtools.adapter;
import android.support.v7.widget.RecyclerView;
import android.view.View;
import com.eyeem.recyclerviewtools.OnItemClickListener;
/**
* Created by budius on 01.04.15.
* <p/>
* Simple implementation of {@link android.widget.AdapterView.OnItemClickListener AdapterView.OnItemClickListener}
* refactored to {@link android.support.v7.widget.RecyclerView RecyclerView}
* <p/>
* Just like original, this only catch clicks on the whole view.
* For finer control on the target view for the click, you still must create a custom implementation.
*/
/* package */ class OnItemClickListenerDetector implements View.OnClickListener {
private final RecyclerView recyclerView;
private final OnItemClickListener onItemClickListener;
final boolean ignoreExtras;
OnItemClickListenerDetector(
RecyclerView recyclerView,
OnItemClickListener onItemClickListener,
boolean ignoreExtras) {
this.recyclerView = recyclerView;
this.onItemClickListener = onItemClickListener;
this.ignoreExtras = ignoreExtras;
}
@Override
public void onClick(View view) {
RecyclerView.ViewHolder holder = recyclerView.getChildViewHolder(view);
int position = holder.getAdapterPosition();
long id = holder.getItemId();
RecyclerView.Adapter adapter = recyclerView.getAdapter();
if (ignoreExtras && adapter instanceof WrapAdapter) {
WrapAdapter a = (WrapAdapter) adapter;
position = a.recyclerToWrappedPosition.get(position);
}
// this can happen if data set is changing onItemClick and user clicks fast
if (position < 0 || position >= adapter.getItemCount()) return;
onItemClickListener.onItemClick(recyclerView, view, position, id, holder);
}
}
| {'content_hash': 'd121f2b06eb0026044323e30ba68d0ae', 'timestamp': '', 'source': 'github', 'line_count': 51, 'max_line_length': 114, 'avg_line_length': 35.470588235294116, 'alnum_prop': 0.7357656163626313, 'repo_name': 'eyeem/RecyclerViewTools', 'id': '1d76d2043cf44e1f946bdd4254b8ce8b6b43d2e6', 'size': '1809', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'library/src/main/java/com/eyeem/recyclerviewtools/adapter/OnItemClickListenerDetector.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Java', 'bytes': '54239'}]} |
package org.kaazing.gateway.service.cluster;
import java.util.Collection;
import java.util.List;
import java.util.concurrent.locks.Lock;
import com.hazelcast.core.IdGenerator;
import org.kaazing.gateway.service.messaging.buffer.MessageBufferFactory;
import org.kaazing.gateway.service.messaging.collections.CollectionsFactory;
public interface ClusterContext {
// initialization
void start();
// for gateway shutdown
void dispose();
// cluster participation
MemberId getLocalMember();
String getInstanceKey(MemberId memberId);
String getClusterName();
// Return a list of the memberIds of all current cluster members
Collection<MemberId> getMemberIds();
List<MemberId> getAccepts();
List<MemberId> getConnects();
ClusterConnectOptionsContext getConnectOptions();
// cluster collections
Lock getLock(Object obj);
IdGenerator getIdGenerator(String name);
// cluster messaging
void addReceiveTopic(String name);
void addReceiveQueue(String name);
<T> T send(Object msg, MemberId member) throws Exception;
<T> T send(Object msg, String name) throws Exception;
void send(Object msg, final SendListener listener, MemberId member);
void send(Object msg, final SendListener listener, String name);
<T> void setReceiver(Class<T> type, ReceiveListener<T> receiveListener);
<T> void removeReceiver(Class<T> type);
// event listener
void addMembershipEventListener(MembershipEventListener eventListener);
void removeMembershipEventListener(MembershipEventListener eventListener);
// instanceKey listener
void addInstanceKeyListener(InstanceKeyListener instanceKeyListener);
void removeInstanceKeyListener(InstanceKeyListener instanceKeyListener);
// balancermap listener
void addBalancerMapListener(BalancerMapListener balancerMapListener);
void removeBalancerMapListener(BalancerMapListener balancerMapListener);
MessageBufferFactory getMessageBufferFactory();
CollectionsFactory getCollectionsFactory();
void logClusterState();
}
| {'content_hash': '3e0340fc604a552d21e90c6fa23e5dd8', 'timestamp': '', 'source': 'github', 'line_count': 64, 'max_line_length': 78, 'avg_line_length': 32.78125, 'alnum_prop': 0.7640610104861774, 'repo_name': 'biddyweb/gateway', 'id': '921cdc3f28e19a42ed8fc680491cff33f873b20e', 'size': '2981', 'binary': False, 'copies': '7', 'ref': 'refs/heads/develop', 'path': 'service/spi/src/main/java/org/kaazing/gateway/service/cluster/ClusterContext.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Batchfile', 'bytes': '2250'}, {'name': 'HTML', 'bytes': '38948'}, {'name': 'Java', 'bytes': '10720288'}, {'name': 'JavaScript', 'bytes': '3868'}, {'name': 'Shell', 'bytes': '5534'}, {'name': 'XSLT', 'bytes': '5493'}]} |
<?php
/*
* TODO: as this file was created from a BLUEPRINT file,
* you may want to change ports, paths and/or methods (e.g. for hub)
* to meet your specific service/server needs
*/
require_module('ui/page');
class BluePrintPage extends ServicePage {
public function __construct(Service $service) {
parent::__construct($service);
$this->addJS('js/blueprint.js');
}
protected function renderRightMenu() {
$links = LinkUI('manager log',
'viewlog.php?sid='.$this->service->getSID())->setExternal(true);
if ($this->service->isRunning()) {
$console_url = $this->service->getAccessLocation();
$links .= ' · ' .LinkUI('hub', $console_url)->setExternal(true);
}
return '<div class="rightmenu">'.$links.'</div>';
}
protected function renderInstanceActions() {
return EditableTag()->setColor('purple')->setID('node')->setValue('0')->setText('BluePrint Nodes');
}
public function renderContent() {
return $this->renderInstancesSection();
}
}
?>
| {'content_hash': 'bebc9cb2a887f09c904012438273c80c', 'timestamp': '', 'source': 'github', 'line_count': 37, 'max_line_length': 107, 'avg_line_length': 29.62162162162162, 'alnum_prop': 0.6076642335766423, 'repo_name': 'ConPaaS-team/conpaas', 'id': '15ec789be8fbe714aa6f691ae19aa487fff7c305', 'size': '2737', 'binary': False, 'copies': '3', 'ref': 'refs/heads/master', 'path': 'conpaas-blueprints/conpaas-frontend/www/lib/ui/page/blueprint/__init__.php', 'mode': '33188', 'license': 'bsd-3-clause', 'language': [{'name': 'ApacheConf', 'bytes': '79'}, {'name': 'Batchfile', 'bytes': '2136'}, {'name': 'C', 'bytes': '12346'}, {'name': 'CSS', 'bytes': '47680'}, {'name': 'HTML', 'bytes': '5494'}, {'name': 'Java', 'bytes': '404303'}, {'name': 'JavaScript', 'bytes': '164519'}, {'name': 'M4', 'bytes': '553'}, {'name': 'Makefile', 'bytes': '78772'}, {'name': 'Nginx', 'bytes': '1980'}, {'name': 'PHP', 'bytes': '1900634'}, {'name': 'Python', 'bytes': '2842443'}, {'name': 'Shell', 'bytes': '232043'}, {'name': 'Smarty', 'bytes': '15450'}]} |
package org.apache.hyracks.control.common.job.profiling.om;
import java.io.DataInput;
import java.io.DataOutput;
import java.io.IOException;
import java.io.Serializable;
import org.apache.hyracks.api.io.IWritable;
import org.apache.hyracks.api.partitions.PartitionId;
import org.apache.hyracks.control.common.job.profiling.counters.MultiResolutionEventProfiler;
public class PartitionProfile implements IWritable, Serializable {
private static final long serialVersionUID = 1L;
private PartitionId pid;
private long openTime;
private long closeTime;
private MultiResolutionEventProfiler mrep;
public static PartitionProfile create(DataInput dis) throws IOException {
PartitionProfile partitionProfile = new PartitionProfile();
partitionProfile.readFields(dis);
return partitionProfile;
}
private PartitionProfile() {
}
public PartitionProfile(PartitionId pid, long openTime, long closeTime, MultiResolutionEventProfiler mrep) {
this.pid = pid;
this.openTime = openTime;
this.closeTime = closeTime;
this.mrep = mrep;
}
public PartitionId getPartitionId() {
return pid;
}
public long getOpenTime() {
return openTime;
}
public long getCloseTime() {
return closeTime;
}
public MultiResolutionEventProfiler getSamples() {
return mrep;
}
@Override
public void writeFields(DataOutput output) throws IOException {
output.writeLong(closeTime);
output.writeLong(openTime);
mrep.writeFields(output);
pid.writeFields(output);
}
@Override
public void readFields(DataInput input) throws IOException {
closeTime = input.readLong();
openTime = input.readLong();
mrep = MultiResolutionEventProfiler.create(input);
pid = PartitionId.create(input);
}
} | {'content_hash': '6d10aa221472af5036271db11282998d', 'timestamp': '', 'source': 'github', 'line_count': 72, 'max_line_length': 112, 'avg_line_length': 26.430555555555557, 'alnum_prop': 0.7004729374671571, 'repo_name': 'kisskys/incubator-asterixdb-hyracks', 'id': 'b1cd16d58f67228830e159253ef64ef05f99a154', 'size': '2710', 'binary': False, 'copies': '3', 'ref': 'refs/heads/master', 'path': 'hyracks/hyracks-control/hyracks-control-common/src/main/java/org/apache/hyracks/control/common/job/profiling/om/PartitionProfile.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Batchfile', 'bytes': '2606'}, {'name': 'CSS', 'bytes': '893'}, {'name': 'HTML', 'bytes': '8762'}, {'name': 'Java', 'bytes': '8406768'}, {'name': 'JavaScript', 'bytes': '24904'}, {'name': 'Shell', 'bytes': '16545'}]} |
// ----------------------------------------------------------------------------
// <copyright file="Enums.cs" company="Exit Games GmbH">
// PhotonNetwork Framework for Unity - Copyright (C) 2011 Exit Games GmbH
// </copyright>
// <summary>
//
// </summary>
// <author>[email protected]</author>
// ----------------------------------------------------------------------------
#pragma warning disable 1587
/// \file
/// <summary>Wraps up several of the commonly used enumerations. </summary>
#pragma warning restore 1587
using System;
using ExitGames.Client.Photon;
/// <summary>
/// This enum defines the set of MonoMessages Photon Unity Networking is using as callbacks. Implemented by PunBehaviour.
/// </summary>
/// <remarks>
/// Much like "Update()" in Unity, PUN will call methods in specific situations.
/// Often, these methods are triggered when network operations complete (example: when joining a room).
///
/// All those methods are defined and described in this enum and implemented by PunBehaviour
/// (which makes it easy to implement them as override).
///
/// Each entry is the name of such a method and the description tells you when it gets used by PUN.
///
/// Make sure to read the remarks per entry as some methods have optional parameters.
/// </remarks>
/// \ingroup publicApi
public enum PhotonNetworkingMessage
{
/// <summary>
/// Called when the initial connection got established but before you can use the server. OnJoinedLobby() or OnConnectedToMaster() are called when PUN is ready.
/// </summary>
/// <remarks>
/// This callback is only useful to detect if the server can be reached at all (technically).
/// Most often, it's enough to implement OnFailedToConnectToPhoton() and OnDisconnectedFromPhoton().
///
/// <i>OnJoinedLobby() or OnConnectedToMaster() are called when PUN is ready.</i>
///
/// When this is called, the low level connection is established and PUN will send your AppId, the user, etc in the background.
/// This is not called for transitions from the masterserver to game servers.
///
/// Example: void OnConnectedToPhoton() { ... }
/// </remarks>
OnConnectedToPhoton,
/// <summary>
/// Called when the local user/client left a room.
/// </summary>
/// <remarks>
/// When leaving a room, PUN brings you back to the Master Server.
/// Before you can use lobbies and join or create rooms, OnJoinedLobby() or OnConnectedToMaster() will get called again.
///
/// Example: void OnLeftRoom() { ... }
/// </remarks>
OnLeftRoom,
/// <summary>
/// Called after switching to a new MasterClient when the current one leaves.
/// </summary>
/// <remarks>
/// This is not called when this client enters a room.
/// The former MasterClient is still in the player list when this method get called.
///
/// Example: void OnMasterClientSwitched(PhotonPlayer newMasterClient) { ... }
/// </remarks>
OnMasterClientSwitched,
/// <summary>
/// Called when a CreateRoom() call failed. Optional parameters provide ErrorCode and message.
/// </summary>
/// <remarks>
/// Most likely because the room name is already in use (some other client was faster than you).
/// PUN logs some info if the PhotonNetwork.logLevel is >= PhotonLogLevel.Informational.
///
/// Example: void OnPhotonCreateRoomFailed() { ... }
///
/// Example: void OnPhotonCreateRoomFailed(object[] codeAndMsg) { // codeAndMsg[0] is short ErrorCode. codeAndMsg[1] is string debug msg. }
/// </remarks>
OnPhotonCreateRoomFailed,
/// <summary>
/// Called when a JoinRoom() call failed. Optional parameters provide ErrorCode and message.
/// </summary>
/// <remarks>
/// Most likely error is that the room does not exist or the room is full (some other client was faster than you).
/// PUN logs some info if the PhotonNetwork.logLevel is >= PhotonLogLevel.Informational.
///
/// Example: void OnPhotonJoinRoomFailed() { ... }
///
/// Example: void OnPhotonJoinRoomFailed(object[] codeAndMsg) { // codeAndMsg[0] is short ErrorCode. codeAndMsg[1] is string debug msg. }
/// </remarks>
OnPhotonJoinRoomFailed,
/// <summary>
/// Called when this client created a room and entered it. OnJoinedRoom() will be called as well.
/// </summary>
/// <remarks>
/// This callback is only called on the client which created a room (see PhotonNetwork.CreateRoom).
///
/// As any client might close (or drop connection) anytime, there is a chance that the
/// creator of a room does not execute OnCreatedRoom.
///
/// If you need specific room properties or a "start signal", it is safer to implement
/// OnMasterClientSwitched() and to make the new MasterClient check the room's state.
///
/// Example: void OnCreatedRoom() { ... }
/// </remarks>
OnCreatedRoom,
/// <summary>
/// Called on entering a lobby on the Master Server. The actual room-list updates will call OnReceivedRoomListUpdate().
/// </summary>
/// <remarks>
/// Note: When PhotonNetwork.autoJoinLobby is false, OnConnectedToMaster() will be called and the room list won't become available.
///
/// While in the lobby, the roomlist is automatically updated in fixed intervals (which you can't modify).
/// The room list gets available when OnReceivedRoomListUpdate() gets called after OnJoinedLobby().
///
/// Example: void OnJoinedLobby() { ... }
/// </remarks>
OnJoinedLobby,
/// <summary>
/// Called after leaving a lobby.
/// </summary>
/// <remarks>
/// When you leave a lobby, [CreateRoom](@ref PhotonNetwork.CreateRoom) and [JoinRandomRoom](@ref PhotonNetwork.JoinRandomRoom)
/// automatically refer to the default lobby.
///
/// Example: void OnLeftLobby() { ... }
/// </remarks>
OnLeftLobby,
/// <summary>
/// Called after disconnecting from the Photon server.
/// </summary>
/// <remarks>
/// In some cases, other callbacks are called before OnDisconnectedFromPhoton is called.
/// Examples: OnConnectionFail() and OnFailedToConnectToPhoton().
///
/// Example: void OnDisconnectedFromPhoton() { ... }
/// </remarks>
OnDisconnectedFromPhoton,
/// <summary>
/// Called when something causes the connection to fail (after it was established), followed by a call to OnDisconnectedFromPhoton().
/// </summary>
/// <remarks>
/// If the server could not be reached in the first place, OnFailedToConnectToPhoton is called instead.
/// The reason for the error is provided as StatusCode.
///
/// Example: void OnConnectionFail(DisconnectCause cause) { ... }
/// </remarks>
OnConnectionFail,
/// <summary>
/// Called if a connect call to the Photon server failed before the connection was established, followed by a call to OnDisconnectedFromPhoton().
/// </summary>
/// <remarks>
/// OnConnectionFail only gets called when a connection to a Photon server was established in the first place.
///
/// Example: void OnFailedToConnectToPhoton(DisconnectCause cause) { ... }
/// </remarks>
OnFailedToConnectToPhoton,
/// <summary>
/// Called for any update of the room-listing while in a lobby (PhotonNetwork.insideLobby) on the Master Server.
/// </summary>
/// <remarks>
/// PUN provides the list of rooms by PhotonNetwork.GetRoomList().<br/>
/// Each item is a RoomInfo which might include custom properties (provided you defined those as lobby-listed when creating a room).
///
/// Not all types of lobbies provide a listing of rooms to the client. Some are silent and specialized for server-side matchmaking.
///
/// Example: void OnReceivedRoomListUpdate() { ... }
/// </remarks>
OnReceivedRoomListUpdate,
/// <summary>
/// Called when entering a room (by creating or joining it). Called on all clients (including the Master Client).
/// </summary>
/// <remarks>
/// This method is commonly used to instantiate player characters.
/// If a match has to be started "actively", you can instead call an [PunRPC](@ref PhotonView.RPC) triggered by a user's button-press or a timer.
///
/// When this is called, you can usually already access the existing players in the room via PhotonNetwork.playerList.
/// Also, all custom properties should be already available as Room.customProperties. Check Room.playerCount to find out if
/// enough players are in the room to start playing.
///
/// Example: void OnJoinedRoom() { ... }
/// </remarks>
OnJoinedRoom,
/// <summary>
/// Called when a remote player entered the room. This PhotonPlayer is already added to the playerlist at this time.
/// </summary>
/// <remarks>
/// If your game starts with a certain number of players, this callback can be useful to check the
/// Room.playerCount and find out if you can start.
///
/// Example: void OnPhotonPlayerConnected(PhotonPlayer newPlayer) { ... }
/// </remarks>
OnPhotonPlayerConnected,
/// <summary>
/// Called when a remote player left the room. This PhotonPlayer is already removed from the playerlist at this time.
/// </summary>
/// <remarks>
/// When your client calls PhotonNetwork.leaveRoom, PUN will call this method on the remaining clients.
/// When a remote client drops connection or gets closed, this callback gets executed. after a timeout
/// of several seconds.
///
/// Example: void OnPhotonPlayerDisconnected(PhotonPlayer otherPlayer) { ... }
/// </remarks>
OnPhotonPlayerDisconnected,
/// <summary>
/// Called after a JoinRandom() call failed. Optional parameters provide ErrorCode and message.
/// </summary>
/// <remarks>
/// Most likely all rooms are full or no rooms are available.
/// When using multiple lobbies (via JoinLobby or TypedLobby), another lobby might have more/fitting rooms.
/// PUN logs some info if the PhotonNetwork.logLevel is >= PhotonLogLevel.Informational.
///
/// Example: void OnPhotonRandomJoinFailed() { ... }
///
/// Example: void OnPhotonRandomJoinFailed(object[] codeAndMsg) { // codeAndMsg[0] is short ErrorCode. codeAndMsg[1] is string debug msg. }
/// </remarks>
OnPhotonRandomJoinFailed,
/// <summary>
/// Called after the connection to the master is established and authenticated but only when PhotonNetwork.autoJoinLobby is false.
/// </summary>
/// <remarks>
/// If you set PhotonNetwork.autoJoinLobby to true, OnJoinedLobby() will be called instead of this.
///
/// You can join rooms and create them even without being in a lobby. The default lobby is used in that case.
/// The list of available rooms won't become available unless you join a lobby via PhotonNetwork.joinLobby.
///
/// Example: void OnConnectedToMaster() { ... }
/// </remarks>
OnConnectedToMaster,
/// <summary>
/// Implement to customize the data a PhotonView regularly synchronizes. Called every 'network-update' when observed by PhotonView.
/// </summary>
/// <remarks>
/// This method will be called in scripts that are assigned as Observed component of a PhotonView.
/// PhotonNetwork.sendRateOnSerialize affects how often this method is called.
/// PhotonNetwork.sendRate affects how often packages are sent by this client.
///
/// Implementing this method, you can customize which data a PhotonView regularly synchronizes.
/// Your code defines what is being sent (content) and how your data is used by receiving clients.
///
/// Unlike other callbacks, <i>OnPhotonSerializeView only gets called when it is assigned
/// to a PhotonView</i> as PhotonView.observed script.
///
/// To make use of this method, the PhotonStream is essential. It will be in "writing" mode" on the
/// client that controls a PhotonView (PhotonStream.isWriting == true) and in "reading mode" on the
/// remote clients that just receive that the controlling client sends.
///
/// If you skip writing any value into the stream, PUN will skip the update. Used carefully, this can
/// conserve bandwidth and messages (which have a limit per room/second).
///
/// Note that OnPhotonSerializeView is not called on remote clients when the sender does not send
/// any update. This can't be used as "x-times per second Update()".
///
/// Example: void OnPhotonSerializeView(PhotonStream stream, PhotonMessageInfo info) { ... }
/// </remarks>
OnPhotonSerializeView,
/// <summary>
/// Called on all scripts on a GameObject (and children) that have been Instantiated using PhotonNetwork.Instantiate.
/// </summary>
/// <remarks>
/// PhotonMessageInfo parameter provides info about who created the object and when (based off PhotonNetworking.time).
///
/// Example: void OnPhotonInstantiate(PhotonMessageInfo info) { ... }
/// </remarks>
OnPhotonInstantiate,
/// <summary>
/// Because the concurrent user limit was (temporarily) reached, this client is rejected by the server and disconnecting.
/// </summary>
/// <remarks>
/// When this happens, the user might try again later. You can't create or join rooms in OnPhotonMaxCcuReached(), cause the client will be disconnecting.
/// You can raise the CCU limits with a new license (when you host yourself) or extended subscription (when using the Photon Cloud).
/// The Photon Cloud will mail you when the CCU limit was reached. This is also visible in the Dashboard (webpage).
///
/// Example: void OnPhotonMaxCccuReached() { ... }
/// </remarks>
OnPhotonMaxCccuReached,
/// <summary>
/// Called when a room's custom properties changed. The propertiesThatChanged contains all that was set via Room.SetCustomProperties.
/// </summary>
/// <remarks>
/// Since v1.25 this method has one parameter: Hashtable propertiesThatChanged.
/// Changing properties must be done by Room.SetCustomProperties, which causes this callback locally, too.
///
/// Example: void OnPhotonCustomRoomPropertiesChanged(Hashtable propertiesThatChanged) { ... }
/// </remarks>
OnPhotonCustomRoomPropertiesChanged,
/// <summary>
/// Called when custom player-properties are changed. Player and the changed properties are passed as object[].
/// </summary>
/// <remarks>
/// Since v1.25 this method has one parameter: object[] playerAndUpdatedProps, which contains two entries.<br/>
/// [0] is the affected PhotonPlayer.<br/>
/// [1] is the Hashtable of properties that changed.<br/>
///
/// We are using a object[] due to limitations of Unity's GameObject.SendMessage (which has only one optional parameter).
///
/// Changing properties must be done by PhotonPlayer.SetCustomProperties, which causes this callback locally, too.
///
/// Example:<pre>
/// void OnPhotonPlayerPropertiesChanged(object[] playerAndUpdatedProps) {
/// PhotonPlayer player = playerAndUpdatedProps[0] as PhotonPlayer;
/// Hashtable props = playerAndUpdatedProps[1] as Hashtable;
/// //...
/// }</pre>
/// </remarks>
OnPhotonPlayerPropertiesChanged,
/// <summary>
/// Called when the server sent the response to a FindFriends request and updated PhotonNetwork.Friends.
/// </summary>
/// <remarks>
/// The friends list is available as PhotonNetwork.Friends, listing name, online state and
/// the room a user is in (if any).
///
/// Example: void OnUpdatedFriendList() { ... }
/// </remarks>
OnUpdatedFriendList,
/// <summary>
/// Called when the custom authentication failed. Followed by disconnect!
/// </summary>
/// <remarks>
/// Custom Authentication can fail due to user-input, bad tokens/secrets.
/// If authentication is successful, this method is not called. Implement OnJoinedLobby() or OnConnectedToMaster() (as usual).
///
/// During development of a game, it might also fail due to wrong configuration on the server side.
/// In those cases, logging the debugMessage is very important.
///
/// Unless you setup a custom authentication service for your app (in the [Dashboard](https://www.photonengine.com/dashboard)),
/// this won't be called!
///
/// Example: void OnCustomAuthenticationFailed(string debugMessage) { ... }
/// </remarks>
OnCustomAuthenticationFailed,
/// <summary>
/// Called when your Custom Authentication service responds with additional data.
/// </summary>
/// <remarks>
/// Custom Authentication services can include some custom data in their response.
/// When present, that data is made available in this callback as Dictionary.
/// While the keys of your data have to be strings, the values can be either string or a number (in Json).
/// You need to make extra sure, that the value type is the one you expect. Numbers become (currently) int64.
///
/// Example: void OnCustomAuthenticationResponse(Dictionary<string, object> data) { ... }
/// </remarks>
/// <see cref="https://doc.photonengine.com/en/realtime/current/reference/custom-authentication"/>
OnCustomAuthenticationResponse,
/// <summary>
/// Called by PUN when the response to a WebRPC is available. See PhotonNetwork.WebRPC.
/// </summary>
/// <remarks>
/// Important: The response.ReturnCode is 0 if Photon was able to reach your web-service.
/// The content of the response is what your web-service sent. You can create a WebResponse instance from it.
/// Example: WebRpcResponse webResponse = new WebRpcResponse(operationResponse);
///
/// Please note: Class OperationResponse is in a namespace which needs to be "used":
/// using ExitGames.Client.Photon; // includes OperationResponse (and other classes)
///
/// The OperationResponse.ReturnCode by Photon is:
/// 0 for "OK"
/// -3 for "Web-Service not configured" (see Dashboard / WebHooks)
/// -5 for "Web-Service does now have RPC path/name" (at least for Azure)
///
/// Example: void OnWebRpcResponse(OperationResponse response) { ... }
/// </remarks>
OnWebRpcResponse,
/// <summary>
/// Called when another player requests ownership of a PhotonView from you (the current owner).
/// </summary>
/// <remarks>
/// The parameter viewAndPlayer contains:
///
/// PhotonView view = viewAndPlayer[0] as PhotonView;
///
/// PhotonPlayer requestingPlayer = viewAndPlayer[1] as PhotonPlayer;
/// </remarks>
/// <example>void OnOwnershipRequest(object[] viewAndPlayer) {} //</example>
OnOwnershipRequest,
/// <summary>
/// Called when the Master Server sent an update for the Lobby Statistics, updating PhotonNetwork.LobbyStatistics.
/// </summary>
/// <remarks>
/// This callback has two preconditions:
/// EnableLobbyStatistics must be set to true, before this client connects.
/// And the client has to be connected to the Master Server, which is providing the info about lobbies.
/// </remarks>
OnLobbyStatisticsUpdate,
/// <summary>
/// Called when a remote Photon Player activity changed. This will be called ONLY is PlayerTtl is greater then 0.
///
/// Use PhotonPlayer.IsInactive to check the current activity state
///
/// Example: void OnPhotonPlayerActivityChanged(PhotonPlayer otherPlayer) {...}
/// </summary>
/// <remarks>
/// This callback has precondition:
/// PlayerTtl must be greater then 0
/// </remarks>
OnPhotonPlayerActivityChanged,
/// <summary>
/// Called when a PhotonView Owner is transfered to a Player.
/// </summary>
/// <remarks>
/// The parameter viewAndPlayers contains:
///
/// PhotonView view = viewAndPlayers[0] as PhotonView;
///
/// PhotonPlayer newOwner = viewAndPlayers[1] as PhotonPlayer;
///
/// PhotonPlayer oldOwner = viewAndPlayers[2] as PhotonPlayer;
/// </remarks>
/// <example>void OnOwnershipTransfered(object[] viewAndPlayers) {} //</example>
OnOwnershipTransfered,
}
/// <summary>Used to define the level of logging output created by the PUN classes. Either log errors, info (some more) or full.</summary>
/// \ingroup publicApi
public enum PhotonLogLevel
{
/// <summary>Show only errors. Minimal output. Note: Some might be "runtime errors" which you have to expect.</summary>
ErrorsOnly,
/// <summary>Logs some of the workflow, calls and results.</summary>
Informational,
/// <summary>Every available log call gets into the console/log. Only use for debugging.</summary>
Full
}
/// <summary>Enum of "target" options for RPCs. These define which remote clients get your RPC call. </summary>
/// \ingroup publicApi
public enum PhotonTargets
{
/// <summary>Sends the RPC to everyone else and executes it immediately on this client. Player who join later will not execute this RPC.</summary>
All,
/// <summary>Sends the RPC to everyone else. This client does not execute the RPC. Player who join later will not execute this RPC.</summary>
Others,
/// <summary>Sends the RPC to MasterClient only. Careful: The MasterClient might disconnect before it executes the RPC and that might cause dropped RPCs.</summary>
MasterClient,
/// <summary>Sends the RPC to everyone else and executes it immediately on this client. New players get the RPC when they join as it's buffered (until this client leaves).</summary>
AllBuffered,
/// <summary>Sends the RPC to everyone. This client does not execute the RPC. New players get the RPC when they join as it's buffered (until this client leaves).</summary>
OthersBuffered,
/// <summary>Sends the RPC to everyone (including this client) through the server.</summary>
/// <remarks>
/// This client executes the RPC like any other when it received it from the server.
/// Benefit: The server's order of sending the RPCs is the same on all clients.
/// </remarks>
AllViaServer,
/// <summary>Sends the RPC to everyone (including this client) through the server and buffers it for players joining later.</summary>
/// <remarks>
/// This client executes the RPC like any other when it received it from the server.
/// Benefit: The server's order of sending the RPCs is the same on all clients.
/// </remarks>
AllBufferedViaServer
}
/// <summary>Currently available <a href="http://doc.photonengine.com/en/pun/current/reference/regions">Photon Cloud regions</a> as enum.</summary>
/// <remarks>
/// This is used in PhotonNetwork.ConnectToRegion.
/// </remarks>
public enum CloudRegionCode
{
/// <summary>European servers in Amsterdam.</summary>
eu = 0,
/// <summary>US servers (East Coast).</summary>
us = 1,
/// <summary>Asian servers in Singapore.</summary>
asia = 2,
/// <summary>Japanese servers in Tokyo.</summary>
jp = 3,
/// <summary>Australian servers in Melbourne.</summary>
au = 5,
///<summary>USA West, San José, usw</summary>
usw = 6,
///<summary>South America, Sao Paulo, sa</summary>
sa = 7,
///<summary>Canada East, Montreal, cae</summary>
cae = 8,
///<summary>South Korea, Seoul, kr</summary>
kr = 9,
///<summary>India, Chennai, in</summary>
@in = 10,
/// <summary>Russia, ru</summary>
ru = 11,
/// <summary>No region selected.</summary>
none = 4
};
/// <summary>
/// Available regions as enum of flags. To be used as "enabled" flags for Best Region pinging.
/// </summary>
/// <remarks>Note that these enum values skip CloudRegionCode.none and their values are in strict order (power of 2).</remarks>
[Flags]
public enum CloudRegionFlag
{
eu = 1 << 0,
us = 1 << 1,
asia = 1 << 2,
jp = 1 << 3,
au = 1 << 4,
usw = 1 << 5,
sa = 1 << 6,
cae = 1 << 7,
kr = 1 << 8,
@in = 1 << 9,
ru = 1 << 10
};
/// <summary>
/// High level connection state of the client. Better use the more detailed <see cref="ClientState"/>.
/// </summary>
public enum ConnectionState
{
Disconnected,
Connecting,
Connected,
Disconnecting,
InitializingApplication
}
/// <summary>
/// Defines how the communication gets encrypted.
/// </summary>
public enum EncryptionMode
{
/// <summary>
/// This is the default encryption mode: Messages get encrypted only on demand (when you send operations with the "encrypt" parameter set to true).
/// </summary>
PayloadEncryption,
/// <summary>
/// With this encryption mode for UDP, the connection gets setup and all further datagrams get encrypted almost entirely. On-demand message encryption (like in PayloadEncryption) is skipped.
/// </summary>
/// <remarks>
/// This mode requires AuthOnce or AuthOnceWss as AuthMode!
/// </remarks>
DatagramEncryption = 10,
}
public static class EncryptionDataParameters
{
/// <summary>
/// Key for encryption mode
/// </summary>
public const byte Mode = 0;
/// <summary>
/// Key for first secret
/// </summary>
public const byte Secret1 = 1;
/// <summary>
/// Key for second secret
/// </summary>
public const byte Secret2 = 2;
} | {'content_hash': '3dac8078c3a3ccd663352f86fb27aa45', 'timestamp': '', 'source': 'github', 'line_count': 594, 'max_line_length': 194, 'avg_line_length': 42.86531986531987, 'alnum_prop': 0.6711570183017831, 'repo_name': 'mark818/VRGame', 'id': '0f68e462b72e5de534ecc4f7025bf9413287b5ee', 'size': '25465', 'binary': False, 'copies': '2', 'ref': 'refs/heads/master', 'path': 'Assets/Photon Unity Networking/Plugins/PhotonNetwork/Enums.cs', 'mode': '33188', 'license': 'bsd-3-clause', 'language': [{'name': 'C#', 'bytes': '2517881'}, {'name': 'HLSL', 'bytes': '11555'}, {'name': 'JavaScript', 'bytes': '43789'}, {'name': 'Mask', 'bytes': '305'}, {'name': 'ShaderLab', 'bytes': '27926'}]} |
<?php
/**
* @runParallel
*/
class UnitTestInSubSubLevelTest extends PHPUnit_Framework_TestCase
{
/**
* @group fixtures
*/
public function testTruth()
{
$this->assertTrue(true);
}
/**
* @group fixtures
*/
public function testFalsehood()
{
$this->assertFalse(false);
}
/**
* @group fixtures
*/
public function testArrayLength()
{
$elems = array(1,2,3,4,5);
$this->assertEquals(5, sizeof($elems));
}
}
| {'content_hash': 'ff6ab174255b36337e2c71021d233a4f', 'timestamp': '', 'source': 'github', 'line_count': 31, 'max_line_length': 66, 'avg_line_length': 16.548387096774192, 'alnum_prop': 0.5341130604288499, 'repo_name': 'quizlet/paratest', 'id': '04a48d88b9018142c2e4d3edeb0fdef88ee245a0', 'size': '513', 'binary': False, 'copies': '5', 'ref': 'refs/heads/master', 'path': 'test/fixtures/passing-tests/level1/level2/UnitTestInSubSubLevelTest.php', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Batchfile', 'bytes': '70'}, {'name': 'PHP', 'bytes': '237507'}, {'name': 'Ruby', 'bytes': '270'}]} |
@interface MyViewController () @end
@implementation MyViewController {}
@synthesize webView, baseURL, url;
- (void)viewDidLoad
{
[super viewDidLoad];
self.title = @"Presented ViewController";
[self.webView setMainFrameURL:[self appURL]];
}
- (IBAction)dismiss:(id)sender
{
if (self.presentingViewController) {
[self.presentingViewController dismissViewController:self];
} else {
// for the 'show' transition
[self.view.window close];
}
}
// Changes the greeting message by executing a function in JavaScript.
// This is triggered from the Change Greeting menu item.
- (IBAction)changeGreeting:(id)sender
{
[[webView windowScriptObject] evaluateWebScript:@"changeGreeting('Hello from Objective-C!')"];
}
// Here we grab the URL to the bundled index.html document.
// Normally it would be the URL to your web app such as @"http://example.com".
- (NSString *)appURL
{
// return [[[NSBundle mainBundle] URLForResource:@"http://www.google" withExtension:@"html"] absoluteString];
// self.baseURL = [NSURL URLWithString:@"file:///path/to/web_root/"];
// self.url = [NSURL URLWithString:@"folder/file.html" relativeToURL:self.baseURL];
NSString *path = [[NSBundle mainBundle] pathForResource:@"index" ofType:@"html"];
self.url = [NSURL fileURLWithPath:path];
[[self.webView mainFrame] loadRequest:[NSURLRequest requestWithURL:self.url]];
NSURL *absURL = [self.url absoluteURL];
NSLog(@"absURL = %@", absURL);
NSString *getURL = [NSString stringWithContentsOfURL:absURL encoding:1000 error:nil];
// self.title = [NSString stringWithContentsOfURL:absURL encoding:1000 error:nil];;
return getURL;
}
// This delegate method gets triggered every time the page loads, but before the JavaScript runs
- (void)webView:(WebView *)webView windowScriptObjectAvailable:(WebScriptObject *)windowScriptObject
{
// Allow this class to be usable through the "window.app" object in JavaScript
// This could be any Objective-C class
[windowScriptObject setValue:self forKey:@"app"];
}
- (IBAction)resetSafari:(id)sender {
[self openAppleScript:(NSString *)@"SafariCloseAllWindows"];
[self openAppleScript:(NSString *)@"ResetSafari"];
}
#pragma mark ViewController openAppleScript
- (void)openAppleScript:(NSString *)scriptName
{
NSLog(@"%@", NSStringFromSelector(_cmd));
NSString *path = [[NSBundle mainBundle] pathForResource:scriptName ofType:@"scpt"];
NSURL *openUrl = [NSURL fileURLWithPath:path]; NSDictionary *errors = [NSDictionary dictionary];
NSAppleScript *appleScript = [[NSAppleScript alloc] initWithContentsOfURL:openUrl error:&errors];
[appleScript executeAndReturnError:nil];
}
- (IBAction)openMyPayPal:(id)sender{
[self openAppleScript:(NSString *)@"OpenMyPaypal"];
}
@end
| {'content_hash': '99e5612dbe476b6b06200030baf9cded', 'timestamp': '', 'source': 'github', 'line_count': 87, 'max_line_length': 110, 'avg_line_length': 31.908045977011493, 'alnum_prop': 0.7312680115273775, 'repo_name': 'RandyMcMillan/GoogleHacks', 'id': '144dd413cccbd97fef34a8b9d66c3d468e7999ca', 'size': '2951', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'osx/GoogleHacks/GoogleHacks/MyViewController.m', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'AppleScript', 'bytes': '1208'}, {'name': 'C', 'bytes': '8953'}, {'name': 'CSS', 'bytes': '10163'}, {'name': 'HTML', 'bytes': '5058'}, {'name': 'JavaScript', 'bytes': '93489'}, {'name': 'Objective-C', 'bytes': '259798'}, {'name': 'REALbasic', 'bytes': '98880'}, {'name': 'Shell', 'bytes': '29131'}]} |
/**
* @author Toru Nagashima
* See LICENSE file in root directory for full license.
*/
"use strict"
const { READ } = require("eslint-utils")
const checkForPreferGlobal = require("../../util/check-prefer-global")
const trackMap = {
globals: {
process: { [READ]: true },
},
modules: {
process: { [READ]: true },
},
}
module.exports = {
meta: {
docs: {
description: 'enforce either `process` or `require("process")`',
category: "Stylistic Issues",
recommended: false,
url:
"https://github.com/mysticatea/eslint-plugin-node/blob/v11.1.0/docs/rules/prefer-global/process.md",
},
type: "suggestion",
fixable: null,
schema: [{ enum: ["always", "never"] }],
messages: {
preferGlobal:
"Unexpected use of 'require(\"process\")'. Use the global variable 'process' instead.",
preferModule:
"Unexpected use of the global variable 'process'. Use 'require(\"process\")' instead.",
},
},
create(context) {
return {
"Program:exit"() {
checkForPreferGlobal(context, trackMap)
},
}
},
}
| {'content_hash': '18574d225594fc58aa63fbcf4f99929f', 'timestamp': '', 'source': 'github', 'line_count': 46, 'max_line_length': 116, 'avg_line_length': 27.282608695652176, 'alnum_prop': 0.5306772908366534, 'repo_name': 'BigBoss424/portfolio', 'id': '05482f756ed77730071cda9a6d69ce7c696c4086', 'size': '1255', 'binary': False, 'copies': '2', 'ref': 'refs/heads/master', 'path': 'v6/node_modules/eslint-plugin-node/lib/rules/prefer-global/process.js', 'mode': '33188', 'license': 'apache-2.0', 'language': []} |
using System;
using System.Diagnostics;
#pragma warning disable 1591
// ReSharper disable UnusedMember.Global
// ReSharper disable MemberCanBePrivate.Global
// ReSharper disable UnusedAutoPropertyAccessor.Global
// ReSharper disable IntroduceOptionalParameters.Global
// ReSharper disable MemberCanBeProtected.Global
// ReSharper disable InconsistentNaming
// ReSharper disable once CheckNamespace
namespace StackingEntities.Model.Annotations
{
/// <summary>
/// Indicates that the value of the marked element could be <c>null</c> sometimes,
/// so the check for <c>null</c> is necessary before its usage
/// </summary>
/// <example><code>
/// [CanBeNull] public object Test() { return null; }
/// public void UseTest() {
/// var p = Test();
/// var s = p.ToString(); // Warning: Possible 'System.NullReferenceException'
/// }
/// </code></example>
[AttributeUsage(
AttributeTargets.Method | AttributeTargets.Parameter | AttributeTargets.Property |
AttributeTargets.Delegate | AttributeTargets.Field | AttributeTargets.Event)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class CanBeNullAttribute : Attribute { }
/// <summary>
/// Indicates that the value of the marked element could never be <c>null</c>
/// </summary>
/// <example><code>
/// [NotNull] public object Foo() {
/// return null; // Warning: Possible 'null' assignment
/// }
/// </code></example>
[AttributeUsage(
AttributeTargets.Method | AttributeTargets.Parameter | AttributeTargets.Property |
AttributeTargets.Delegate | AttributeTargets.Field | AttributeTargets.Event)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class NotNullAttribute : Attribute { }
/// <summary>
/// Indicates that collection or enumerable value does not contain null elements
/// </summary>
[AttributeUsage(
AttributeTargets.Method | AttributeTargets.Parameter | AttributeTargets.Property |
AttributeTargets.Delegate | AttributeTargets.Field)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class ItemNotNullAttribute : Attribute { }
/// <summary>
/// Indicates that collection or enumerable value can contain null elements
/// </summary>
[AttributeUsage(
AttributeTargets.Method | AttributeTargets.Parameter | AttributeTargets.Property |
AttributeTargets.Delegate | AttributeTargets.Field)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class ItemCanBeNullAttribute : Attribute { }
/// <summary>
/// Indicates that the marked method builds string by format pattern and (optional) arguments.
/// Parameter, which contains format string, should be given in constructor. The format string
/// should be in <see cref="string.Format(IFormatProvider,string,object[])"/>-like form
/// </summary>
/// <example><code>
/// [StringFormatMethod("message")]
/// public void ShowError(string message, params object[] args) { /* do something */ }
/// public void Foo() {
/// ShowError("Failed: {0}"); // Warning: Non-existing argument in format string
/// }
/// </code></example>
[AttributeUsage(
AttributeTargets.Constructor | AttributeTargets.Method | AttributeTargets.Delegate)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class StringFormatMethodAttribute : Attribute
{
/// <param name="formatParameterName">
/// Specifies which parameter of an annotated method should be treated as format-string
/// </param>
public StringFormatMethodAttribute(string formatParameterName)
{
FormatParameterName = formatParameterName;
}
public string FormatParameterName { get; private set; }
}
/// <summary>
/// For a parameter that is expected to be one of the limited set of values.
/// Specify fields of which type should be used as values for this parameter.
/// </summary>
[AttributeUsage(AttributeTargets.Parameter | AttributeTargets.Property | AttributeTargets.Field)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class ValueProviderAttribute : Attribute
{
public ValueProviderAttribute(string name)
{
Name = name;
}
[NotNull] public string Name { get; private set; }
}
/// <summary>
/// Indicates that the function argument should be string literal and match one
/// of the parameters of the caller function. For example, ReSharper annotates
/// the parameter of <see cref="System.ArgumentNullException"/>
/// </summary>
/// <example><code>
/// public void Foo(string param) {
/// if (param == null)
/// throw new ArgumentNullException("par"); // Warning: Cannot resolve symbol
/// }
/// </code></example>
[AttributeUsage(AttributeTargets.Parameter)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class InvokerParameterNameAttribute : Attribute { }
/// <summary>
/// Indicates that the method is contained in a type that implements
/// <c>System.ComponentModel.INotifyPropertyChanged</c> interface and this method
/// is used to notify that some property value changed
/// </summary>
/// <remarks>
/// The method should be non-static and conform to one of the supported signatures:
/// <list>
/// <item><c>NotifyChanged(string)</c></item>
/// <item><c>NotifyChanged(params string[])</c></item>
/// <item><c>NotifyChanged{T}(Expression{Func{T}})</c></item>
/// <item><c>NotifyChanged{T,U}(Expression{Func{T,U}})</c></item>
/// <item><c>SetProperty{T}(ref T, T, string)</c></item>
/// </list>
/// </remarks>
/// <example><code>
/// public class Foo : INotifyPropertyChanged {
/// public event PropertyChangedEventHandler PropertyChanged;
/// [NotifyPropertyChangedInvocator]
/// protected virtual void NotifyChanged(string propertyName) { ... }
///
/// private string _name;
/// public string Name {
/// get { return _name; }
/// set { _name = value; NotifyChanged("LastName"); /* Warning */ }
/// }
/// }
/// </code>
/// Examples of generated notifications:
/// <list>
/// <item><c>NotifyChanged("Property")</c></item>
/// <item><c>NotifyChanged(() => Property)</c></item>
/// <item><c>NotifyChanged((VM x) => x.Property)</c></item>
/// <item><c>SetProperty(ref myField, value, "Property")</c></item>
/// </list>
/// </example>
[AttributeUsage(AttributeTargets.Method)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class NotifyPropertyChangedInvocatorAttribute : Attribute
{
public NotifyPropertyChangedInvocatorAttribute() { }
public NotifyPropertyChangedInvocatorAttribute(string parameterName)
{
ParameterName = parameterName;
}
public string ParameterName { get; private set; }
}
/// <summary>
/// Describes dependency between method input and output
/// </summary>
/// <syntax>
/// <p>Function Definition Table syntax:</p>
/// <list>
/// <item>FDT ::= FDTRow [;FDTRow]*</item>
/// <item>FDTRow ::= Input => Output | Output <= Input</item>
/// <item>Input ::= ParameterName: Value [, Input]*</item>
/// <item>Output ::= [ParameterName: Value]* {halt|stop|void|nothing|Value}</item>
/// <item>Value ::= true | false | null | notnull | canbenull</item>
/// </list>
/// If method has single input parameter, it's name could be omitted.<br/>
/// Using <c>halt</c> (or <c>void</c>/<c>nothing</c>, which is the same)
/// for method output means that the methos doesn't return normally.<br/>
/// <c>canbenull</c> annotation is only applicable for output parameters.<br/>
/// You can use multiple <c>[ContractAnnotation]</c> for each FDT row,
/// or use single attribute with rows separated by semicolon.<br/>
/// </syntax>
/// <examples><list>
/// <item><code>
/// [ContractAnnotation("=> halt")]
/// public void TerminationMethod()
/// </code></item>
/// <item><code>
/// [ContractAnnotation("halt <= condition: false")]
/// public void Assert(bool condition, string text) // regular assertion method
/// </code></item>
/// <item><code>
/// [ContractAnnotation("s:null => true")]
/// public bool IsNullOrEmpty(string s) // string.IsNullOrEmpty()
/// </code></item>
/// <item><code>
/// // A method that returns null if the parameter is null,
/// // and not null if the parameter is not null
/// [ContractAnnotation("null => null; notnull => notnull")]
/// public object Transform(object data)
/// </code></item>
/// <item><code>
/// [ContractAnnotation("s:null=>false; =>true,result:notnull; =>false, result:null")]
/// public bool TryParse(string s, out Person result)
/// </code></item>
/// </list></examples>
[AttributeUsage(AttributeTargets.Method, AllowMultiple = true)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class ContractAnnotationAttribute : Attribute
{
public ContractAnnotationAttribute([NotNull] string contract)
: this(contract, false) { }
public ContractAnnotationAttribute([NotNull] string contract, bool forceFullStates)
{
Contract = contract;
ForceFullStates = forceFullStates;
}
public string Contract { get; private set; }
public bool ForceFullStates { get; private set; }
}
/// <summary>
/// Indicates that marked element should be localized or not
/// </summary>
/// <example><code>
/// [LocalizationRequiredAttribute(true)]
/// public class Foo {
/// private string str = "my string"; // Warning: Localizable string
/// }
/// </code></example>
[AttributeUsage(AttributeTargets.All)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class LocalizationRequiredAttribute : Attribute
{
public LocalizationRequiredAttribute() : this(true) { }
public LocalizationRequiredAttribute(bool required)
{
Required = required;
}
public bool Required { get; private set; }
}
/// <summary>
/// Indicates that the value of the marked type (or its derivatives)
/// cannot be compared using '==' or '!=' operators and <c>Equals()</c>
/// should be used instead. However, using '==' or '!=' for comparison
/// with <c>null</c> is always permitted.
/// </summary>
/// <example><code>
/// [CannotApplyEqualityOperator]
/// class NoEquality { }
/// class UsesNoEquality {
/// public void Test() {
/// var ca1 = new NoEquality();
/// var ca2 = new NoEquality();
/// if (ca1 != null) { // OK
/// bool condition = ca1 == ca2; // Warning
/// }
/// }
/// }
/// </code></example>
[AttributeUsage(
AttributeTargets.Interface | AttributeTargets.Class | AttributeTargets.Struct)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class CannotApplyEqualityOperatorAttribute : Attribute { }
/// <summary>
/// When applied to a target attribute, specifies a requirement for any type marked
/// with the target attribute to implement or inherit specific type or types.
/// </summary>
/// <example><code>
/// [BaseTypeRequired(typeof(IComponent)] // Specify requirement
/// public class ComponentAttribute : Attribute { }
/// [Component] // ComponentAttribute requires implementing IComponent interface
/// public class MyComponent : IComponent { }
/// </code></example>
[AttributeUsage(AttributeTargets.Class, AllowMultiple = true)]
[BaseTypeRequired(typeof(Attribute))]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class BaseTypeRequiredAttribute : Attribute
{
public BaseTypeRequiredAttribute([NotNull] Type baseType)
{
BaseType = baseType;
}
[NotNull] public Type BaseType { get; private set; }
}
/// <summary>
/// Indicates that the marked symbol is used implicitly
/// (e.g. via reflection, in external library), so this symbol
/// will not be marked as unused (as well as by other usage inspections)
/// </summary>
[AttributeUsage(AttributeTargets.All)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class UsedImplicitlyAttribute : Attribute
{
public UsedImplicitlyAttribute()
: this(ImplicitUseKindFlags.Default, ImplicitUseTargetFlags.Default) { }
public UsedImplicitlyAttribute(ImplicitUseKindFlags useKindFlags)
: this(useKindFlags, ImplicitUseTargetFlags.Default) { }
public UsedImplicitlyAttribute(ImplicitUseTargetFlags targetFlags)
: this(ImplicitUseKindFlags.Default, targetFlags) { }
public UsedImplicitlyAttribute(
ImplicitUseKindFlags useKindFlags, ImplicitUseTargetFlags targetFlags)
{
UseKindFlags = useKindFlags;
TargetFlags = targetFlags;
}
public ImplicitUseKindFlags UseKindFlags { get; private set; }
public ImplicitUseTargetFlags TargetFlags { get; private set; }
}
/// <summary>
/// Should be used on attributes and causes ReSharper
/// to not mark symbols marked with such attributes as unused
/// (as well as by other usage inspections)
/// </summary>
[AttributeUsage(AttributeTargets.Class | AttributeTargets.GenericParameter)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class MeansImplicitUseAttribute : Attribute
{
public MeansImplicitUseAttribute()
: this(ImplicitUseKindFlags.Default, ImplicitUseTargetFlags.Default) { }
public MeansImplicitUseAttribute(ImplicitUseKindFlags useKindFlags)
: this(useKindFlags, ImplicitUseTargetFlags.Default) { }
public MeansImplicitUseAttribute(ImplicitUseTargetFlags targetFlags)
: this(ImplicitUseKindFlags.Default, targetFlags) { }
public MeansImplicitUseAttribute(
ImplicitUseKindFlags useKindFlags, ImplicitUseTargetFlags targetFlags)
{
UseKindFlags = useKindFlags;
TargetFlags = targetFlags;
}
[UsedImplicitly] public ImplicitUseKindFlags UseKindFlags { get; private set; }
[UsedImplicitly] public ImplicitUseTargetFlags TargetFlags { get; private set; }
}
[Flags]
public enum ImplicitUseKindFlags
{
Default = Access | Assign | InstantiatedWithFixedConstructorSignature,
/// <summary>Only entity marked with attribute considered used</summary>
Access = 1,
/// <summary>Indicates implicit assignment to a member</summary>
Assign = 2,
/// <summary>
/// Indicates implicit instantiation of a type with fixed constructor signature.
/// That means any unused constructor parameters won't be reported as such.
/// </summary>
InstantiatedWithFixedConstructorSignature = 4,
/// <summary>Indicates implicit instantiation of a type</summary>
InstantiatedNoFixedConstructorSignature = 8,
}
/// <summary>
/// Specify what is considered used implicitly when marked
/// with <see cref="MeansImplicitUseAttribute"/> or <see cref="UsedImplicitlyAttribute"/>
/// </summary>
[Flags]
public enum ImplicitUseTargetFlags
{
Default = Itself,
Itself = 1,
/// <summary>Members of entity marked with attribute are considered used</summary>
Members = 2,
/// <summary>Entity marked with attribute and all its members considered used</summary>
WithMembers = Itself | Members
}
/// <summary>
/// This attribute is intended to mark publicly available API
/// which should not be removed and so is treated as used
/// </summary>
[MeansImplicitUse]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class PublicAPIAttribute : Attribute
{
public PublicAPIAttribute() { }
public PublicAPIAttribute([NotNull] string comment)
{
Comment = comment;
}
public string Comment { get; private set; }
}
/// <summary>
/// Tells code analysis engine if the parameter is completely handled
/// when the invoked method is on stack. If the parameter is a delegate,
/// indicates that delegate is executed while the method is executed.
/// If the parameter is an enumerable, indicates that it is enumerated
/// while the method is executed
/// </summary>
[AttributeUsage(AttributeTargets.Parameter)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class InstantHandleAttribute : Attribute { }
/// <summary>
/// Indicates that a method does not make any observable state changes.
/// The same as <c>System.Diagnostics.Contracts.PureAttribute</c>
/// </summary>
/// <example><code>
/// [Pure] private int Multiply(int x, int y) { return x * y; }
/// public void Foo() {
/// const int a = 2, b = 2;
/// Multiply(a, b); // Waring: Return value of pure method is not used
/// }
/// </code></example>
[AttributeUsage(AttributeTargets.Method)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class PureAttribute : Attribute { }
/// <summary>
/// Indicates that a parameter is a path to a file or a folder within a web project.
/// Path can be relative or absolute, starting from web root (~)
/// </summary>
[AttributeUsage(AttributeTargets.Parameter)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public class PathReferenceAttribute : Attribute
{
public PathReferenceAttribute() { }
public PathReferenceAttribute([PathReference] string basePath)
{
BasePath = basePath;
}
public string BasePath { get; private set; }
}
[AttributeUsage(AttributeTargets.Assembly, AllowMultiple = true)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class AspMvcAreaMasterLocationFormatAttribute : Attribute
{
public AspMvcAreaMasterLocationFormatAttribute(string format)
{
Format = format;
}
public string Format { get; private set; }
}
[AttributeUsage(AttributeTargets.Assembly, AllowMultiple = true)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class AspMvcAreaPartialViewLocationFormatAttribute : Attribute
{
public AspMvcAreaPartialViewLocationFormatAttribute(string format)
{
Format = format;
}
public string Format { get; private set; }
}
[AttributeUsage(AttributeTargets.Assembly, AllowMultiple = true)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class AspMvcAreaViewLocationFormatAttribute : Attribute
{
public AspMvcAreaViewLocationFormatAttribute(string format)
{
Format = format;
}
public string Format { get; private set; }
}
[AttributeUsage(AttributeTargets.Assembly, AllowMultiple = true)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class AspMvcMasterLocationFormatAttribute : Attribute
{
public AspMvcMasterLocationFormatAttribute(string format)
{
Format = format;
}
public string Format { get; private set; }
}
[AttributeUsage(AttributeTargets.Assembly, AllowMultiple = true)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class AspMvcPartialViewLocationFormatAttribute : Attribute
{
public AspMvcPartialViewLocationFormatAttribute(string format)
{
Format = format;
}
public string Format { get; private set; }
}
[AttributeUsage(AttributeTargets.Assembly, AllowMultiple = true)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class AspMvcViewLocationFormatAttribute : Attribute
{
public AspMvcViewLocationFormatAttribute(string format)
{
Format = format;
}
public string Format { get; private set; }
}
/// <summary>
/// ASP.NET MVC attribute. If applied to a parameter, indicates that the parameter
/// is an MVC action. If applied to a method, the MVC action name is calculated
/// implicitly from the context. Use this attribute for custom wrappers similar to
/// <c>System.Web.Mvc.Html.ChildActionExtensions.RenderAction(HtmlHelper, String)</c>
/// </summary>
[AttributeUsage(AttributeTargets.Parameter | AttributeTargets.Method)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class AspMvcActionAttribute : Attribute
{
public AspMvcActionAttribute() { }
public AspMvcActionAttribute(string anonymousProperty)
{
AnonymousProperty = anonymousProperty;
}
public string AnonymousProperty { get; private set; }
}
/// <summary>
/// ASP.NET MVC attribute. Indicates that a parameter is an MVC area.
/// Use this attribute for custom wrappers similar to
/// <c>System.Web.Mvc.Html.ChildActionExtensions.RenderAction(HtmlHelper, String)</c>
/// </summary>
[AttributeUsage(AttributeTargets.Parameter)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class AspMvcAreaAttribute : PathReferenceAttribute
{
public AspMvcAreaAttribute() { }
public AspMvcAreaAttribute(string anonymousProperty)
{
AnonymousProperty = anonymousProperty;
}
public string AnonymousProperty { get; private set; }
}
/// <summary>
/// ASP.NET MVC attribute. If applied to a parameter, indicates that the parameter is
/// an MVC controller. If applied to a method, the MVC controller name is calculated
/// implicitly from the context. Use this attribute for custom wrappers similar to
/// <c>System.Web.Mvc.Html.ChildActionExtensions.RenderAction(HtmlHelper, String, String)</c>
/// </summary>
[AttributeUsage(AttributeTargets.Parameter | AttributeTargets.Method)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class AspMvcControllerAttribute : Attribute
{
public AspMvcControllerAttribute() { }
public AspMvcControllerAttribute(string anonymousProperty)
{
AnonymousProperty = anonymousProperty;
}
public string AnonymousProperty { get; private set; }
}
/// <summary>
/// ASP.NET MVC attribute. Indicates that a parameter is an MVC Master. Use this attribute
/// for custom wrappers similar to <c>System.Web.Mvc.Controller.View(String, String)</c>
/// </summary>
[AttributeUsage(AttributeTargets.Parameter)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class AspMvcMasterAttribute : Attribute { }
/// <summary>
/// ASP.NET MVC attribute. Indicates that a parameter is an MVC model type. Use this attribute
/// for custom wrappers similar to <c>System.Web.Mvc.Controller.View(String, Object)</c>
/// </summary>
[AttributeUsage(AttributeTargets.Parameter)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class AspMvcModelTypeAttribute : Attribute { }
/// <summary>
/// ASP.NET MVC attribute. If applied to a parameter, indicates that the parameter is an MVC
/// partial view. If applied to a method, the MVC partial view name is calculated implicitly
/// from the context. Use this attribute for custom wrappers similar to
/// <c>System.Web.Mvc.Html.RenderPartialExtensions.RenderPartial(HtmlHelper, String)</c>
/// </summary>
[AttributeUsage(AttributeTargets.Parameter | AttributeTargets.Method)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class AspMvcPartialViewAttribute : PathReferenceAttribute { }
/// <summary>
/// ASP.NET MVC attribute. Allows disabling inspections for MVC views within a class or a method
/// </summary>
[AttributeUsage(AttributeTargets.Class | AttributeTargets.Method)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class AspMvcSupressViewErrorAttribute : Attribute { }
/// <summary>
/// ASP.NET MVC attribute. Indicates that a parameter is an MVC display template.
/// Use this attribute for custom wrappers similar to
/// <c>System.Web.Mvc.Html.DisplayExtensions.DisplayForModel(HtmlHelper, String)</c>
/// </summary>
[AttributeUsage(AttributeTargets.Parameter)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class AspMvcDisplayTemplateAttribute : Attribute { }
/// <summary>
/// ASP.NET MVC attribute. Indicates that a parameter is an MVC editor template.
/// Use this attribute for custom wrappers similar to
/// <c>System.Web.Mvc.Html.EditorExtensions.EditorForModel(HtmlHelper, String)</c>
/// </summary>
[AttributeUsage(AttributeTargets.Parameter)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class AspMvcEditorTemplateAttribute : Attribute { }
/// <summary>
/// ASP.NET MVC attribute. Indicates that a parameter is an MVC template.
/// Use this attribute for custom wrappers similar to
/// <c>System.ComponentModel.DataAnnotations.UIHintAttribute(System.String)</c>
/// </summary>
[AttributeUsage(AttributeTargets.Parameter)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class AspMvcTemplateAttribute : Attribute { }
/// <summary>
/// ASP.NET MVC attribute. If applied to a parameter, indicates that the parameter
/// is an MVC view. If applied to a method, the MVC view name is calculated implicitly
/// from the context. Use this attribute for custom wrappers similar to
/// <c>System.Web.Mvc.Controller.View(Object)</c>
/// </summary>
[AttributeUsage(AttributeTargets.Parameter | AttributeTargets.Method)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class AspMvcViewAttribute : PathReferenceAttribute { }
/// <summary>
/// ASP.NET MVC attribute. When applied to a parameter of an attribute,
/// indicates that this parameter is an MVC action name
/// </summary>
/// <example><code>
/// [ActionName("Foo")]
/// public ActionResult Login(string returnUrl) {
/// ViewBag.ReturnUrl = Url.Action("Foo"); // OK
/// return RedirectToAction("Bar"); // Error: Cannot resolve action
/// }
/// </code></example>
[AttributeUsage(AttributeTargets.Parameter | AttributeTargets.Property)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class AspMvcActionSelectorAttribute : Attribute { }
[AttributeUsage(
AttributeTargets.Parameter | AttributeTargets.Property | AttributeTargets.Field)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class HtmlElementAttributesAttribute : Attribute
{
public HtmlElementAttributesAttribute() { }
public HtmlElementAttributesAttribute(string name)
{
Name = name;
}
public string Name { get; private set; }
}
[AttributeUsage(
AttributeTargets.Parameter | AttributeTargets.Field | AttributeTargets.Property)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class HtmlAttributeValueAttribute : Attribute
{
public HtmlAttributeValueAttribute([NotNull] string name)
{
Name = name;
}
[NotNull] public string Name { get; private set; }
}
/// <summary>
/// Razor attribute. Indicates that a parameter or a method is a Razor section.
/// Use this attribute for custom wrappers similar to
/// <c>System.Web.WebPages.WebPageBase.RenderSection(String)</c>
/// </summary>
[AttributeUsage(AttributeTargets.Parameter | AttributeTargets.Method)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class RazorSectionAttribute : Attribute { }
/// <summary>
/// Indicates how method invocation affects content of the collection
/// </summary>
[AttributeUsage(AttributeTargets.Method)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class CollectionAccessAttribute : Attribute
{
public CollectionAccessAttribute(CollectionAccessType collectionAccessType)
{
CollectionAccessType = collectionAccessType;
}
public CollectionAccessType CollectionAccessType { get; private set; }
}
[Flags]
public enum CollectionAccessType
{
/// <summary>Method does not use or modify content of the collection</summary>
None = 0,
/// <summary>Method only reads content of the collection but does not modify it</summary>
Read = 1,
/// <summary>Method can change content of the collection but does not add new elements</summary>
ModifyExistingContent = 2,
/// <summary>Method can add new elements to the collection</summary>
UpdatedContent = ModifyExistingContent | 4
}
/// <summary>
/// Indicates that the marked method is assertion method, i.e. it halts control flow if
/// one of the conditions is satisfied. To set the condition, mark one of the parameters with
/// <see cref="AssertionConditionAttribute"/> attribute
/// </summary>
[AttributeUsage(AttributeTargets.Method)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class AssertionMethodAttribute : Attribute { }
/// <summary>
/// Indicates the condition parameter of the assertion method. The method itself should be
/// marked by <see cref="AssertionMethodAttribute"/> attribute. The mandatory argument of
/// the attribute is the assertion type.
/// </summary>
[AttributeUsage(AttributeTargets.Parameter)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class AssertionConditionAttribute : Attribute
{
public AssertionConditionAttribute(AssertionConditionType conditionType)
{
ConditionType = conditionType;
}
public AssertionConditionType ConditionType { get; private set; }
}
/// <summary>
/// Specifies assertion type. If the assertion method argument satisfies the condition,
/// then the execution continues. Otherwise, execution is assumed to be halted
/// </summary>
public enum AssertionConditionType
{
/// <summary>Marked parameter should be evaluated to true</summary>
IS_TRUE = 0,
/// <summary>Marked parameter should be evaluated to false</summary>
IS_FALSE = 1,
/// <summary>Marked parameter should be evaluated to null value</summary>
IS_NULL = 2,
/// <summary>Marked parameter should be evaluated to not null value</summary>
IS_NOT_NULL = 3,
}
/// <summary>
/// Indicates that the marked method unconditionally terminates control flow execution.
/// For example, it could unconditionally throw exception
/// </summary>
[Obsolete("Use [ContractAnnotation('=> halt')] instead")]
[AttributeUsage(AttributeTargets.Method)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class TerminatesProgramAttribute : Attribute { }
/// <summary>
/// Indicates that method is pure LINQ method, with postponed enumeration (like Enumerable.Select,
/// .Where). This annotation allows inference of [InstantHandle] annotation for parameters
/// of delegate type by analyzing LINQ method chains.
/// </summary>
[AttributeUsage(AttributeTargets.Method)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class LinqTunnelAttribute : Attribute { }
/// <summary>
/// Indicates that IEnumerable, passed as parameter, is not enumerated.
/// </summary>
[AttributeUsage(AttributeTargets.Parameter)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class NoEnumerationAttribute : Attribute { }
/// <summary>
/// XAML attribute. Indicates the type that has <c>ItemsSource</c> property and should be
/// treated as <c>ItemsControl</c>-derived type, to enable inner items <c>DataContext</c>
/// type resolve.
/// </summary>
[AttributeUsage(AttributeTargets.Class)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class XamlItemsControlAttribute : Attribute { }
/// <summary>
/// XAML attibute. Indicates the property of some <c>BindingBase</c>-derived type, that
/// is used to bind some item of <c>ItemsControl</c>-derived type. This annotation will
/// enable the <c>DataContext</c> type resolve for XAML bindings for such properties.
/// </summary>
/// <remarks>
/// Property should have the tree ancestor of the <c>ItemsControl</c> type or
/// marked with the <see cref="XamlItemsControlAttribute"/> attribute.
/// </remarks>
[AttributeUsage(AttributeTargets.Property)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class XamlItemBindingOfItemsControlAttribute : Attribute { }
[AttributeUsage(AttributeTargets.Class, AllowMultiple = true)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public class AspChildControlTypeAttribute : Attribute
{
public AspChildControlTypeAttribute(string tagName, Type controlType)
{
TagName = tagName;
ControlType = controlType;
}
public string TagName { get; private set; }
public Type ControlType { get; private set; }
}
[AttributeUsage(AttributeTargets.Property | AttributeTargets.Method)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public class AspDataFieldAttribute : Attribute { }
[AttributeUsage(AttributeTargets.Property | AttributeTargets.Method)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public class AspDataFieldsAttribute : Attribute { }
[AttributeUsage(AttributeTargets.Property)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public class AspMethodPropertyAttribute : Attribute { }
[AttributeUsage(AttributeTargets.Class, AllowMultiple = true)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public class AspRequiredAttributeAttribute : Attribute
{
public AspRequiredAttributeAttribute([NotNull] string attribute)
{
Attribute = attribute;
}
public string Attribute { get; private set; }
}
[AttributeUsage(AttributeTargets.Property)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public class AspTypePropertyAttribute : Attribute
{
public bool CreateConstructorReferences { get; private set; }
public AspTypePropertyAttribute(bool createConstructorReferences)
{
CreateConstructorReferences = createConstructorReferences;
}
}
[AttributeUsage(AttributeTargets.Assembly, AllowMultiple = true)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class RazorImportNamespaceAttribute : Attribute
{
public RazorImportNamespaceAttribute(string name)
{
Name = name;
}
public string Name { get; private set; }
}
[AttributeUsage(AttributeTargets.Method)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class RazorHelperCommonAttribute : Attribute { }
[AttributeUsage(AttributeTargets.Property)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class RazorLayoutAttribute : Attribute { }
[AttributeUsage(AttributeTargets.Method)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class RazorWriteLiteralMethodAttribute : Attribute { }
[AttributeUsage(AttributeTargets.Method)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class RazorWriteMethodAttribute : Attribute { }
[AttributeUsage(AttributeTargets.Parameter)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class RazorWriteMethodParameterAttribute : Attribute { }
/// <summary>
/// Indicates that parameter is regular expression pattern.
/// </summary>
[AttributeUsage(AttributeTargets.Parameter)]
[Conditional("JETBRAINS_ANNOTATIONS")]
public sealed class RegexPatternAttribute : Attribute { }
} | {'content_hash': '5971dcb4d1b4e83d0efc46f35bf6e02c', 'timestamp': '', 'source': 'github', 'line_count': 906, 'max_line_length': 100, 'avg_line_length': 37.67439293598234, 'alnum_prop': 0.7113936659537691, 'repo_name': 'Sidneys1/MinecraftMultitool', 'id': 'ea30d7cff35bf1fc06f85081954a6d11e0e98a7c', 'size': '34135', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'StackingEntities.Model/Properties/Annotations.cs', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'C#', 'bytes': '298077'}]} |
<?xml version="1.0" encoding="utf-8"?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en" lang="en">
<head>
<title>Class</title>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8" />
<link rel="stylesheet" href="../css/reset.css" type="text/css" media="screen" />
<link rel="stylesheet" href="../css/main.css" type="text/css" media="screen" />
<link rel="stylesheet" href="../css/github.css" type="text/css" media="screen" />
<script src="../js/jquery-1.3.2.min.js" type="text/javascript" charset="utf-8"></script>
<script src="../js/jquery-effect.js" type="text/javascript" charset="utf-8"></script>
<script src="../js/main.js" type="text/javascript" charset="utf-8"></script>
<script src="../js/highlight.pack.js" type="text/javascript" charset="utf-8"></script>
</head>
<body>
<div class="banner">
<span>Ruby on Rails 4.1.8</span><br />
<h1>
<span class="type">Class</span>
Class
<span class="parent"><
<a href="Object.html">Object</a>
</span>
</h1>
<ul class="files">
<li><a href="../files/__/_rvm/gems/ruby-2_2_0/gems/activesupport-4_1_8/lib/active_support/core_ext/class/attribute_rb.html">/home/kristof/.rvm/gems/ruby-2.2.0/gems/activesupport-4.1.8/lib/active_support/core_ext/class/attribute.rb</a></li>
<li><a href="../files/__/_rvm/gems/ruby-2_2_0/gems/activesupport-4_1_8/lib/active_support/core_ext/class/delegating_attributes_rb.html">/home/kristof/.rvm/gems/ruby-2.2.0/gems/activesupport-4.1.8/lib/active_support/core_ext/class/delegating_attributes.rb</a></li>
<li><a href="../files/__/_rvm/gems/ruby-2_2_0/gems/activesupport-4_1_8/lib/active_support/core_ext/class/subclasses_rb.html">/home/kristof/.rvm/gems/ruby-2.2.0/gems/activesupport-4.1.8/lib/active_support/core_ext/class/subclasses.rb</a></li>
</ul>
</div>
<div id="bodyContent">
<div id="content">
<!-- Method ref -->
<div class="sectiontitle">Methods</div>
<dl class="methods">
<dt>C</dt>
<dd>
<ul>
<li>
<a href="#method-i-class_attribute">class_attribute</a>
</li>
</ul>
</dd>
<dt>S</dt>
<dd>
<ul>
<li>
<a href="#method-i-subclasses">subclasses</a>,
</li>
<li>
<a href="#method-i-superclass_delegating_accessor">superclass_delegating_accessor</a>
</li>
</ul>
</dd>
</dl>
<!-- Methods -->
<div class="sectiontitle">Instance Public methods</div>
<div class="method">
<div class="title method-title" id="method-i-class_attribute">
<b>class_attribute</b>(*attrs)
<a href="../classes/Class.html#method-i-class_attribute" name="method-i-class_attribute" class="permalink">Link</a>
</div>
<div class="description">
<p>Declare a class-level attribute whose value is inheritable by subclasses.
Subclasses can change their own value and it will not impact parent class.</p>
<pre><code>class Base
class_attribute :setting
end
class Subclass < Base
end
Base.setting = true
Subclass.setting # => true
Subclass.setting = false
Subclass.setting # => false
Base.setting # => true
</code></pre>
<p>In the above case as long as Subclass does not assign a value to setting by
performing <code>Subclass.setting = <em>something</em> </code>,
<code>Subclass.setting</code> would read value assigned to parent class.
Once Subclass assigns a value then the value assigned by Subclass would be
returned.</p>
<p>This matches normal Ruby method inheritance: think of writing an attribute
on a subclass as overriding the reader method. However, you need to be
aware when using <code>class_attribute</code> with mutable structures as
<code>Array</code> or <code>Hash</code>. In such cases, you don't want
to do changes in places but use setters:</p>
<pre><code>Base.setting = []
Base.setting # => []
Subclass.setting # => []
# Appending in child changes both parent and child because it is the same object:
Subclass.setting << :foo
Base.setting # => [:foo]
Subclass.setting # => [:foo]
# Use setters to not propagate changes:
Base.setting = []
Subclass.setting += [:foo]
Base.setting # => []
Subclass.setting # => [:foo]
</code></pre>
<p>For convenience, an instance predicate method is defined as well. To skip
it, pass <code>instance_predicate: false</code>.</p>
<pre><code>Subclass.setting? # => false
</code></pre>
<p>Instances may overwrite the class value in the same way:</p>
<pre><code>Base.setting = true
object = Base.new
object.setting # => true
object.setting = false
object.setting # => false
Base.setting # => true
</code></pre>
<p>To opt out of the instance reader method, pass <code>instance_reader:
false</code>.</p>
<pre><code>object.setting # => NoMethodError
object.setting? # => NoMethodError
</code></pre>
<p>To opt out of the instance writer method, pass <code>instance_writer:
false</code>.</p>
<pre><code>object.setting = false # => NoMethodError
</code></pre>
<p>To opt out of both instance methods, pass <code>instance_accessor:
false</code>.</p>
</div>
<div class="sourcecode">
<p class="source-link">
Source:
<a href="javascript:toggleSource('method-i-class_attribute_source')" id="l_method-i-class_attribute_source">show</a>
</p>
<div id="method-i-class_attribute_source" class="dyn-source">
<pre><span class="ruby-comment"># File ../.rvm/gems/ruby-2.2.0/gems/activesupport-4.1.8/lib/active_support/core_ext/class/attribute.rb, line 71</span>
<span class="ruby-keyword">def</span> <span class="ruby-keyword ruby-title">class_attribute</span>(<span class="ruby-operator">*</span><span class="ruby-identifier">attrs</span>)
<span class="ruby-identifier">options</span> = <span class="ruby-identifier">attrs</span>.<span class="ruby-identifier">extract_options!</span>
<span class="ruby-identifier">instance_reader</span> = <span class="ruby-identifier">options</span>.<span class="ruby-identifier">fetch</span>(<span class="ruby-value">:instance_accessor</span>, <span class="ruby-keyword">true</span>) <span class="ruby-operator">&&</span> <span class="ruby-identifier">options</span>.<span class="ruby-identifier">fetch</span>(<span class="ruby-value">:instance_reader</span>, <span class="ruby-keyword">true</span>)
<span class="ruby-identifier">instance_writer</span> = <span class="ruby-identifier">options</span>.<span class="ruby-identifier">fetch</span>(<span class="ruby-value">:instance_accessor</span>, <span class="ruby-keyword">true</span>) <span class="ruby-operator">&&</span> <span class="ruby-identifier">options</span>.<span class="ruby-identifier">fetch</span>(<span class="ruby-value">:instance_writer</span>, <span class="ruby-keyword">true</span>)
<span class="ruby-identifier">instance_predicate</span> = <span class="ruby-identifier">options</span>.<span class="ruby-identifier">fetch</span>(<span class="ruby-value">:instance_predicate</span>, <span class="ruby-keyword">true</span>)
<span class="ruby-identifier">attrs</span>.<span class="ruby-identifier">each</span> <span class="ruby-keyword">do</span> <span class="ruby-operator">|</span><span class="ruby-identifier">name</span><span class="ruby-operator">|</span>
<span class="ruby-identifier">define_singleton_method</span>(<span class="ruby-identifier">name</span>) { <span class="ruby-keyword">nil</span> }
<span class="ruby-identifier">define_singleton_method</span>(<span class="ruby-node">"#{name}?"</span>) { <span class="ruby-operator">!</span><span class="ruby-operator">!</span><span class="ruby-identifier">public_send</span>(<span class="ruby-identifier">name</span>) } <span class="ruby-keyword">if</span> <span class="ruby-identifier">instance_predicate</span>
<span class="ruby-identifier">ivar</span> = <span class="ruby-node">"@#{name}"</span>
<span class="ruby-identifier">define_singleton_method</span>(<span class="ruby-node">"#{name}="</span>) <span class="ruby-keyword">do</span> <span class="ruby-operator">|</span><span class="ruby-identifier">val</span><span class="ruby-operator">|</span>
<span class="ruby-identifier">singleton_class</span>.<span class="ruby-identifier">class_eval</span> <span class="ruby-keyword">do</span>
<span class="ruby-identifier">remove_possible_method</span>(<span class="ruby-identifier">name</span>)
<span class="ruby-identifier">define_method</span>(<span class="ruby-identifier">name</span>) { <span class="ruby-identifier">val</span> }
<span class="ruby-keyword">end</span>
<span class="ruby-keyword">if</span> <span class="ruby-identifier">singleton_class?</span>
<span class="ruby-identifier">class_eval</span> <span class="ruby-keyword">do</span>
<span class="ruby-identifier">remove_possible_method</span>(<span class="ruby-identifier">name</span>)
<span class="ruby-identifier">define_method</span>(<span class="ruby-identifier">name</span>) <span class="ruby-keyword">do</span>
<span class="ruby-keyword">if</span> <span class="ruby-identifier">instance_variable_defined?</span> <span class="ruby-identifier">ivar</span>
<span class="ruby-identifier">instance_variable_get</span> <span class="ruby-identifier">ivar</span>
<span class="ruby-keyword">else</span>
<span class="ruby-identifier">singleton_class</span>.<span class="ruby-identifier">send</span> <span class="ruby-identifier">name</span>
<span class="ruby-keyword">end</span>
<span class="ruby-keyword">end</span>
<span class="ruby-keyword">end</span>
<span class="ruby-keyword">end</span>
<span class="ruby-identifier">val</span>
<span class="ruby-keyword">end</span>
<span class="ruby-keyword">if</span> <span class="ruby-identifier">instance_reader</span>
<span class="ruby-identifier">remove_possible_method</span> <span class="ruby-identifier">name</span>
<span class="ruby-identifier">define_method</span>(<span class="ruby-identifier">name</span>) <span class="ruby-keyword">do</span>
<span class="ruby-keyword">if</span> <span class="ruby-identifier">instance_variable_defined?</span>(<span class="ruby-identifier">ivar</span>)
<span class="ruby-identifier">instance_variable_get</span> <span class="ruby-identifier">ivar</span>
<span class="ruby-keyword">else</span>
<span class="ruby-keyword">self</span>.<span class="ruby-identifier">class</span>.<span class="ruby-identifier">public_send</span> <span class="ruby-identifier">name</span>
<span class="ruby-keyword">end</span>
<span class="ruby-keyword">end</span>
<span class="ruby-identifier">define_method</span>(<span class="ruby-node">"#{name}?"</span>) { <span class="ruby-operator">!</span><span class="ruby-operator">!</span><span class="ruby-identifier">public_send</span>(<span class="ruby-identifier">name</span>) } <span class="ruby-keyword">if</span> <span class="ruby-identifier">instance_predicate</span>
<span class="ruby-keyword">end</span>
<span class="ruby-identifier">attr_writer</span> <span class="ruby-identifier">name</span> <span class="ruby-keyword">if</span> <span class="ruby-identifier">instance_writer</span>
<span class="ruby-keyword">end</span>
<span class="ruby-keyword">end</span></pre>
</div>
</div>
</div>
<div class="method">
<div class="title method-title" id="method-i-subclasses">
<b>subclasses</b>()
<a href="../classes/Class.html#method-i-subclasses" name="method-i-subclasses" class="permalink">Link</a>
</div>
<div class="description">
<p>Returns an array with the direct children of <code>self</code>.</p>
<pre><code>Integer.subclasses # => [Fixnum, Bignum]
class Foo; end
class Bar < Foo; end
class Baz < Bar; end
Foo.subclasses # => [Bar]
</code></pre>
</div>
<div class="sourcecode">
<p class="source-link">
Source:
<a href="javascript:toggleSource('method-i-subclasses_source')" id="l_method-i-subclasses_source">show</a>
</p>
<div id="method-i-subclasses_source" class="dyn-source">
<pre><span class="ruby-comment"># File ../.rvm/gems/ruby-2.2.0/gems/activesupport-4.1.8/lib/active_support/core_ext/class/subclasses.rb, line 35</span>
<span class="ruby-keyword">def</span> <span class="ruby-keyword ruby-title">subclasses</span>
<span class="ruby-identifier">subclasses</span>, <span class="ruby-identifier">chain</span> = [], <span class="ruby-identifier">descendants</span>
<span class="ruby-identifier">chain</span>.<span class="ruby-identifier">each</span> <span class="ruby-keyword">do</span> <span class="ruby-operator">|</span><span class="ruby-identifier">k</span><span class="ruby-operator">|</span>
<span class="ruby-identifier">subclasses</span> <span class="ruby-operator"><<</span> <span class="ruby-identifier">k</span> <span class="ruby-keyword">unless</span> <span class="ruby-identifier">chain</span>.<span class="ruby-identifier">any?</span> { <span class="ruby-operator">|</span><span class="ruby-identifier">c</span><span class="ruby-operator">|</span> <span class="ruby-identifier">c</span> <span class="ruby-operator">></span> <span class="ruby-identifier">k</span> }
<span class="ruby-keyword">end</span>
<span class="ruby-identifier">subclasses</span>
<span class="ruby-keyword">end</span></pre>
</div>
</div>
</div>
<div class="method">
<div class="title method-title" id="method-i-superclass_delegating_accessor">
<b>superclass_delegating_accessor</b>(name, options = {})
<a href="../classes/Class.html#method-i-superclass_delegating_accessor" name="method-i-superclass_delegating_accessor" class="permalink">Link</a>
</div>
<div class="description">
</div>
<div class="sourcecode">
<p class="source-link">
Source:
<a href="javascript:toggleSource('method-i-superclass_delegating_accessor_source')" id="l_method-i-superclass_delegating_accessor_source">show</a>
</p>
<div id="method-i-superclass_delegating_accessor_source" class="dyn-source">
<pre><span class="ruby-comment"># File ../.rvm/gems/ruby-2.2.0/gems/activesupport-4.1.8/lib/active_support/core_ext/class/delegating_attributes.rb, line 5</span>
<span class="ruby-keyword">def</span> <span class="ruby-keyword ruby-title">superclass_delegating_accessor</span>(<span class="ruby-identifier">name</span>, <span class="ruby-identifier">options</span> = {})
<span class="ruby-comment"># Create private _name and _name= methods that can still be used if the public</span>
<span class="ruby-comment"># methods are overridden.</span>
<span class="ruby-identifier">_superclass_delegating_accessor</span>(<span class="ruby-node">"_#{name}"</span>, <span class="ruby-identifier">options</span>)
<span class="ruby-comment"># Generate the public methods name, name=, and name?.</span>
<span class="ruby-comment"># These methods dispatch to the private _name, and _name= methods, making them</span>
<span class="ruby-comment"># overridable.</span>
<span class="ruby-identifier">singleton_class</span>.<span class="ruby-identifier">send</span>(<span class="ruby-value">:define_method</span>, <span class="ruby-identifier">name</span>) { <span class="ruby-identifier">send</span>(<span class="ruby-node">"_#{name}"</span>) }
<span class="ruby-identifier">singleton_class</span>.<span class="ruby-identifier">send</span>(<span class="ruby-value">:define_method</span>, <span class="ruby-node">"#{name}?"</span>) { <span class="ruby-operator">!</span><span class="ruby-operator">!</span><span class="ruby-identifier">send</span>(<span class="ruby-node">"_#{name}"</span>) }
<span class="ruby-identifier">singleton_class</span>.<span class="ruby-identifier">send</span>(<span class="ruby-value">:define_method</span>, <span class="ruby-node">"#{name}="</span>) { <span class="ruby-operator">|</span><span class="ruby-identifier">value</span><span class="ruby-operator">|</span> <span class="ruby-identifier">send</span>(<span class="ruby-node">"_#{name}="</span>, <span class="ruby-identifier">value</span>) }
<span class="ruby-comment"># If an instance_reader is needed, generate public instance methods name and name?.</span>
<span class="ruby-keyword">if</span> <span class="ruby-identifier">options</span>[<span class="ruby-value">:instance_reader</span>] <span class="ruby-operator">!=</span> <span class="ruby-keyword">false</span>
<span class="ruby-identifier">define_method</span>(<span class="ruby-identifier">name</span>) { <span class="ruby-identifier">send</span>(<span class="ruby-node">"_#{name}"</span>) }
<span class="ruby-identifier">define_method</span>(<span class="ruby-node">"#{name}?"</span>) { <span class="ruby-operator">!</span><span class="ruby-operator">!</span><span class="ruby-identifier">send</span>(<span class="ruby-node">"#{name}"</span>) }
<span class="ruby-keyword">end</span>
<span class="ruby-keyword">end</span></pre>
</div>
</div>
</div>
</div>
</div>
</body>
</html> | {'content_hash': '70b72cfb262e58a9070a311e29611ac4', 'timestamp': '', 'source': 'github', 'line_count': 378, 'max_line_length': 493, 'avg_line_length': 50.55291005291005, 'alnum_prop': 0.62033596734523, 'repo_name': 'kristoferrobin/p2p', 'id': '0e6aba409bb7dad702c95b3e19a0a2a0d83ce133', 'size': '19109', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'doc/api/classes/Class.html', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '1033'}, {'name': 'CoffeeScript', 'bytes': '422'}, {'name': 'HTML', 'bytes': '5432'}, {'name': 'JavaScript', 'bytes': '664'}, {'name': 'Ruby', 'bytes': '24321'}]} |
<?php
namespace ZFTest\Rest;
use PHPUnit_Framework_TestCase as TestCase;
use Zend\Http\Request as HttpRequest;
use Zend\InputFilter\InputFilter;
use Zend\Mvc\Router\RouteMatch;
use Zend\Stdlib\Parameters;
use ZF\MvcAuth\Identity\GuestIdentity;
use ZF\Rest\ResourceEvent;
class ResourceEventTest extends TestCase
{
public function setUp()
{
$this->matches = new RouteMatch(array(
'foo' => 'bar',
'baz' => 'inga',
));
$this->query = new Parameters(array(
'foo' => 'bar',
'baz' => 'inga',
));
$this->event = new ResourceEvent();
}
public function testRouteMatchIsNullByDefault()
{
$this->assertNull($this->event->getRouteMatch());
}
public function testQueryParamsAreNullByDefault()
{
$this->assertNull($this->event->getQueryParams());
}
public function testRouteMatchIsMutable()
{
$this->event->setRouteMatch($this->matches);
$this->assertSame($this->matches, $this->event->getRouteMatch());
return $this->event;
}
public function testQueryParamsAreMutable()
{
$this->event->setQueryParams($this->query);
$this->assertSame($this->query, $this->event->getQueryParams());
return $this->event;
}
public function testRequestIsNullByDefault()
{
$this->assertNull($this->event->getRequest());
}
public function testRequestIsMutable()
{
$request = new HttpRequest();
$this->event->setRequest($request);
$this->assertSame($request, $this->event->getRequest());
return $this->event;
}
/**
* @depends testRouteMatchIsMutable
*/
public function testRouteMatchIsNullable(ResourceEvent $event)
{
$event->setRouteMatch(null);
$this->assertNull($event->getRouteMatch());
}
/**
* @depends testQueryParamsAreMutable
*/
public function testQueryParamsAreNullable(ResourceEvent $event)
{
$event->setQueryParams(null);
$this->assertNull($event->getQueryParams());
}
/**
* @depends testRequestIsMutable
*/
public function testRequestIsNullable(ResourceEvent $event)
{
$event->setRequest(null);
$this->assertNull($event->getRequest());
}
public function testCanInjectRequestViaSetParams()
{
$request = new HttpRequest();
$this->event->setParams(array('request' => $request));
$this->assertSame($request, $this->event->getRequest());
}
public function testCanFetchIndividualRouteParameter()
{
$this->event->setRouteMatch($this->matches);
$this->assertEquals('bar', $this->event->getRouteParam('foo'));
$this->assertEquals('inga', $this->event->getRouteParam('baz'));
}
public function testCanFetchIndividualQueryParameter()
{
$this->event->setQueryParams($this->query);
$this->assertEquals('bar', $this->event->getQueryParam('foo'));
$this->assertEquals('inga', $this->event->getQueryParam('baz'));
}
public function testReturnsDefaultParameterWhenPullingUnknownRouteParameter()
{
$this->assertNull($this->event->getRouteParam('foo'));
$this->assertEquals('bat', $this->event->getRouteParam('baz', 'bat'));
}
public function testReturnsDefaultParameterWhenPullingUnknownQueryParameter()
{
$this->assertNull($this->event->getQueryParam('foo'));
$this->assertEquals('bat', $this->event->getQueryParam('baz', 'bat'));
}
public function testInputFilterIsUndefinedByDefault()
{
$this->assertNull($this->event->getInputFilter());
}
/**
* @depends testInputFilterIsUndefinedByDefault
*/
public function testCanComposeInputFilter()
{
$inputFilter = new InputFilter();
$this->event->setInputFilter($inputFilter);
$this->assertSame($inputFilter, $this->event->getInputFilter());
}
/**
* @depends testCanComposeInputFilter
*/
public function testCanNullifyInputFilter()
{
$this->event->setInputFilter(null);
$this->assertNull($this->event->getInputFilter());
}
public function testIdentityIsUndefinedByDefault()
{
$this->assertNull($this->event->getIdentity());
}
/**
* @depends testIdentityIsUndefinedByDefault
*/
public function testCanComposeIdentity()
{
$identity = new GuestIdentity();
$this->event->setIdentity($identity);
$this->assertSame($identity, $this->event->getIdentity());
}
/**
* @depends testCanComposeIdentity
*/
public function testCanNullifyIdentity()
{
$this->event->setIdentity(null);
$this->assertNull($this->event->getIdentity());
}
}
| {'content_hash': '57c599ca684af99478622a283893fb6f', 'timestamp': '', 'source': 'github', 'line_count': 174, 'max_line_length': 81, 'avg_line_length': 27.804597701149426, 'alnum_prop': 0.6242248863166597, 'repo_name': 'nocvp/zf-rest', 'id': '3d3a6c0814ca8925fcb6a3fb7b864b16d86184fa', 'size': '4998', 'binary': False, 'copies': '5', 'ref': 'refs/heads/master', 'path': 'test/ResourceEventTest.php', 'mode': '33188', 'license': 'bsd-3-clause', 'language': [{'name': 'PHP', 'bytes': '211249'}]} |
package search
import (
"encoding/json"
"errors"
"fmt"
"github.com/sebas7dk/go-recipes/config"
"github.com/sebas7dk/go-recipes/models"
elastigo "github.com/mattbaird/elastigo/lib"
)
type Connection struct {
Conn *elastigo.Conn
}
var index string
//SetIndex set the index name
func SetIndex(i string) {
index = i
}
//NewConnection create a new Elastic Search connection
func NewConnection() (*Connection, error) {
c := elastigo.NewConn()
if c == nil {
return nil, errors.New("Unable to connect to Elastic Search")
}
c.Domain = config.Get("ES_DOMAIN")
c.Port = config.Get("ES_PORT")
conn := &Connection{Conn: c}
return conn, nil
}
//Show all the docs in the index
func (c *Connection) Show() ([]models.Recipe, error) {
searchJSON := `{
"query" : {
"match_all" : {}
}
}`
o, err := c.Conn.Search(index, "recipe", nil, searchJSON)
r := BuildResults(o.Hits.Hits)
return r, err
}
//GetById show the doc by id
func (c *Connection) GetById(id string) (*models.Recipe, error) {
var recipe *models.Recipe
o, err := c.Conn.Get(index, "recipe", id, nil)
if err == nil {
json.Unmarshal(*o.Source, &recipe)
recipe.Id = o.Id
}
return recipe, err
}
//Create a new doc
func (c *Connection) Create(r models.Recipe) (elastigo.BaseResponse, error) {
return c.Conn.Index(index, "recipe", "", nil, r)
}
//Update a doc by id
func (c *Connection) Update(id string, r models.Recipe) (elastigo.BaseResponse, error) {
return c.Conn.Index(index, "recipe", id, nil, r)
}
//Query the index and match the search term
func (c *Connection) Query(s string) ([]models.Recipe, error) {
searchJSON := fmt.Sprintf(`{
"query" : {
"multi_match": {
"query" : "%s",
"fields" : ["title^50", "category^30", "instructions^25", "ingredients^20"]
}
}
}`, s)
o, err := c.Conn.Search(index, "recipe", nil, searchJSON)
r := BuildResults(o.Hits.Hits)
return r, err
}
//Delete a doc from the index
func (c *Connection) Delete(id string) (elastigo.BaseResponse, error) {
return c.Conn.Delete(index, "recipe", id, nil)
}
//DeleteIndex alll docs from the index
func (c *Connection) DeleteIndex() (elastigo.BaseResponse, error) {
return c.Conn.DeleteIndex(index)
}
//BuildResults loop through the hits based on the total hits
func BuildResults(recipes []elastigo.Hit) []models.Recipe {
var recipe models.Recipe
rs := make(models.Recipes, 0)
for _, r := range recipes {
if err := json.Unmarshal(*r.Source, &recipe); err == nil {
recipe.Id = r.Id
rs = append(rs, recipe)
}
}
return rs
}
| {'content_hash': '69827d11b5629dfd1a9c8eae8f826f3f', 'timestamp': '', 'source': 'github', 'line_count': 117, 'max_line_length': 88, 'avg_line_length': 22.102564102564102, 'alnum_prop': 0.6550657385924207, 'repo_name': 'sebas7dk/go-recipes', 'id': '1630cef66c4fa5730b0e0761ecea66022a7846a5', 'size': '2586', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'search/search.go', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Go', 'bytes': '12353'}, {'name': 'Makefile', 'bytes': '277'}]} |
(function() {
var ArrayIterator, IteratorOutput, PairwiseArrayIterator, __assert_unfinished__, __throw_iterator_finished__;
__throw_iterator_finished__ = function() {
throw new Error("Iterator has already finished");
};
__assert_unfinished__ = function(iteratorOutput) {
if (iteratorOutput.done) {
return __throw_iterator_finished__();
}
};
IteratorOutput = (function() {
function IteratorOutput(value) {
this.value = value;
this.done = false;
}
return IteratorOutput;
})();
ArrayIterator = (function() {
function ArrayIterator(array) {
this.array = array;
this.index = 0;
this.out = new IteratorOutput;
}
ArrayIterator.prototype.next = function() {
var array, index, out;
array = this.array, index = this.index, out = this.out;
__assert_unfinished__(out);
if (index < array.length) {
out.value = array[index];
} else {
out.value = void 0;
out.done = true;
}
this.index += 1;
return out;
};
return ArrayIterator;
})();
PairwiseArrayIterator = (function() {
function PairwiseArrayIterator(array) {
this.array = array;
if (array.length % 2) {
throw new Error("Odd number of elements");
}
this.index = 0;
this.out = new IteratorOutput([]);
}
PairwiseArrayIterator.prototype.next = function() {
var array, index, out;
array = this.array, index = this.index, out = this.out;
__assert_unfinished__(out);
if (index < array.length) {
out.value[0] = array[index];
out.value[1] = array[index + 1];
} else {
out.value[0] = void 0;
out.value[1] = void 0;
out.done = true;
}
this.index += 2;
return out;
};
return PairwiseArrayIterator;
})();
module.exports = {
ArrayIterator: ArrayIterator,
PairwiseArrayIterator: PairwiseArrayIterator
};
}).call(this);
| {'content_hash': 'dd30bf201b9e83f436ec53e5dfd58a61', 'timestamp': '', 'source': 'github', 'line_count': 84, 'max_line_length': 111, 'avg_line_length': 23.678571428571427, 'alnum_prop': 0.5811965811965812, 'repo_name': 'nickfargo/pim', 'id': '5104b9cd1fe9faaea965347c580d7c37e3c8142f', 'size': '2024', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'lib/array-iterators.js', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Shell', 'bytes': '471'}]} |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>projective-geometry: Not compatible 👼</title>
<link rel="shortcut icon" type="image/png" href="../../../../../favicon.png" />
<link href="../../../../../bootstrap.min.css" rel="stylesheet">
<link href="../../../../../bootstrap-custom.css" rel="stylesheet">
<link href="//maxcdn.bootstrapcdn.com/font-awesome/4.2.0/css/font-awesome.min.css" rel="stylesheet">
<script src="../../../../../moment.min.js"></script>
<!-- HTML5 Shim and Respond.js IE8 support of HTML5 elements and media queries -->
<!-- WARNING: Respond.js doesn't work if you view the page via file:// -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/html5shiv/3.7.2/html5shiv.min.js"></script>
<script src="https://oss.maxcdn.com/respond/1.4.2/respond.min.js"></script>
<![endif]-->
</head>
<body>
<div class="container">
<div class="navbar navbar-default" role="navigation">
<div class="container-fluid">
<div class="navbar-header">
<a class="navbar-brand" href="../../../../.."><i class="fa fa-lg fa-flag-checkered"></i> Coq bench</a>
</div>
<div id="navbar" class="collapse navbar-collapse">
<ul class="nav navbar-nav">
<li><a href="../..">clean / released</a></li>
<li class="active"><a href="">8.8.1 / projective-geometry - 8.5.0</a></li>
</ul>
</div>
</div>
</div>
<div class="article">
<div class="row">
<div class="col-md-12">
<a href="../..">« Up</a>
<h1>
projective-geometry
<small>
8.5.0
<span class="label label-info">Not compatible 👼</span>
</small>
</h1>
<p>📅 <em><script>document.write(moment("2022-04-08 16:37:15 +0000", "YYYY-MM-DD HH:mm:ss Z").fromNow());</script> (2022-04-08 16:37:15 UTC)</em><p>
<h2>Context</h2>
<pre># Packages matching: installed
# Name # Installed # Synopsis
base-bigarray base
base-threads base
base-unix base
camlp5 7.14 Preprocessor-pretty-printer of OCaml
conf-findutils 1 Virtual package relying on findutils
conf-perl 2 Virtual package relying on perl
coq 8.8.1 Formal proof management system
num 1.4 The legacy Num library for arbitrary-precision integer and rational arithmetic
ocaml 4.09.1 The OCaml compiler (virtual package)
ocaml-base-compiler 4.09.1 Official release 4.09.1
ocaml-config 1 OCaml Switch Configuration
ocamlfind 1.9.3 A library manager for OCaml
# opam file:
opam-version: "2.0"
maintainer: "[email protected]"
homepage: "https://github.com/coq-contribs/projective-geometry"
license: "Proprietary"
build: [make "-j%{jobs}%"]
install: [make "install"]
remove: ["rm" "-R" "%{lib}%/coq/user-contrib/ProjectiveGeometry"]
depends: [
"ocaml"
"coq" {>= "8.5" & < "8.6~"}
]
tags: [
"keyword:geometry"
"keyword:projective"
"keyword:Fano"
"keyword:homogeneous coordinates model"
"keyword:flat"
"keyword:rank"
"keyword:Desargues"
"keyword:Moulton"
"category:Mathematics/Geometry/General"
"date:2009-10"
]
authors: [ "Nicolas Magaud <[email protected]>" "Julien Narboux <[email protected]>" "Pascal Schreck <[email protected]>" ]
bug-reports: "https://github.com/coq-contribs/projective-geometry/issues"
dev-repo: "git+https://github.com/coq-contribs/projective-geometry.git"
synopsis: "Projective Geometry"
description: """
This contributions contains elements of formalization of projective geometry.
In the plane:
Two axiom systems are shown equivalent. We prove some results about the
decidability of the the incidence and equality predicates. The classic
notion of duality between points and lines is formalized thanks to a
functor. The notion of 'flat' is defined and flats are characterized.
Fano's plane, the smallest projective plane is defined. We show that Fano's plane is desarguesian.
In the space:
We prove Desargues' theorem."""
flags: light-uninstall
url {
src:
"https://github.com/coq-contribs/projective-geometry/archive/v8.5.0.tar.gz"
checksum: "md5=b538b6b3caec10362391436a1f91d9c9"
}
</pre>
<h2>Lint</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Dry install 🏜️</h2>
<p>Dry install with the current Coq version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam install -y --show-action coq-projective-geometry.8.5.0 coq.8.8.1</code></dd>
<dt>Return code</dt>
<dd>5120</dd>
<dt>Output</dt>
<dd><pre>[NOTE] Package coq is already installed (current version is 8.8.1).
The following dependencies couldn't be met:
- coq-projective-geometry -> coq < 8.6~ -> ocaml < 4.06.0
base of this switch (use `--unlock-base' to force)
No solution found, exiting
</pre></dd>
</dl>
<p>Dry install without Coq/switch base, to test if the problem was incompatibility with the current Coq/OCaml version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam remove -y coq; opam install -y --show-action --unlock-base coq-projective-geometry.8.5.0</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Install dependencies</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>0 s</dd>
</dl>
<h2>Install 🚀</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>0 s</dd>
</dl>
<h2>Installation size</h2>
<p>No files were installed.</p>
<h2>Uninstall 🧹</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Missing removes</dt>
<dd>
none
</dd>
<dt>Wrong removes</dt>
<dd>
none
</dd>
</dl>
</div>
</div>
</div>
<hr/>
<div class="footer">
<p class="text-center">
Sources are on <a href="https://github.com/coq-bench">GitHub</a> © Guillaume Claret 🐣
</p>
</div>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
<script src="../../../../../bootstrap.min.js"></script>
</body>
</html>
| {'content_hash': '43f99f6705bde8fc87362a78d175e415', 'timestamp': '', 'source': 'github', 'line_count': 183, 'max_line_length': 228, 'avg_line_length': 42.295081967213115, 'alnum_prop': 0.5683462532299741, 'repo_name': 'coq-bench/coq-bench.github.io', 'id': 'f19569dc00d6c5044e14382b0c17dcdc35b073be', 'size': '7765', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'clean/Linux-x86_64-4.09.1-2.0.6/released/8.8.1/projective-geometry/8.5.0.html', 'mode': '33188', 'license': 'mit', 'language': []} |
@interface DemoViewController ()
@end
@implementation DemoViewController
- (void)viewDidLoad {
[super viewDidLoad];
}
- (void)didReceiveMemoryWarning {
[super didReceiveMemoryWarning];
}
- (IBAction)openCalendar:(id)sender {
[self openCalendarVC:self];
}
- (void)openCalendarVC:(UIViewController *)vc {
if (UI_USER_INTERFACE_IDIOM() == UIUserInterfaceIdiomPad) {
UIViewController *viewController = [[UIStoryboard storyboardWithName:@"main-iPad" bundle:NULL] instantiateViewControllerWithIdentifier:@"MainNav"];
viewController.modalTransitionStyle = UIModalTransitionStyleCoverVertical;
[vc presentViewController:viewController animated:YES completion:nil];
} else {
UIViewController *viewController = [[UIStoryboard storyboardWithName:@"main-iPhone" bundle:NULL] instantiateViewControllerWithIdentifier:@"MainNav"];
viewController.modalTransitionStyle = UIModalTransitionStyleCoverVertical;
[vc presentViewController:viewController animated:YES completion:nil];
}
}
@end
| {'content_hash': 'f9a06995cf8dd0234ef62d7a3c671a5f', 'timestamp': '', 'source': 'github', 'line_count': 35, 'max_line_length': 157, 'avg_line_length': 30.571428571428573, 'alnum_prop': 0.7439252336448599, 'repo_name': 'george-zergy/Calendar', 'id': 'f30b4bcd91848d3d3216afcc6ff06dd941ef9209', 'size': '1251', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'Demo/Other/DemoViewController.m', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Objective-C', 'bytes': '551753'}, {'name': 'Ruby', 'bytes': '1577'}]} |
package club.zhcs.thunder.controller.admin.log;
import org.apache.shiro.authz.annotation.RequiresRoles;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import club.zhcs.thunder.biz.log.LoginLogService;
import club.zhcs.thunder.controller.base.BaseController;
import club.zhcs.thunder.domain.log.LoginLog;
import club.zhcs.titans.utils.db.Pager;
import club.zhcs.titans.utils.db.Result;
/**
*
* @author admin
*
* @email [email protected]
*
*/
@Controller
@RequestMapping("login")
public class LoginLogController extends BaseController {
@Autowired
LoginLogService loginLogService;
@RequestMapping("list")
@RequiresRoles("admin")
public String list(@RequestParam(value = "page", defaultValue = "1") int page, Model model) {
Pager<LoginLog> pager = loginLogService.searchByPage(_fixPage(page));
pager.setUrl(_base() + "/login/list");
model.addAttribute("obj", Result.success().addData("pager", pager).setTitle(" 登录日志列表"));
return "pages/log/login/list";
}
@RequestMapping("search")
@RequiresRoles("admin")
public String search(@RequestParam(value = "page", defaultValue = "1") int page, @RequestParam("key") String key, Model model) {
Pager<LoginLog> pager = loginLogService.searchByKeyAndPage(_fixSearchKey(key), _fixPage(page), "account", "ip");
pager.setUrl(_base() + "/login/search");
pager.addParas("key", key);
model.addAttribute("obj", Result.success().addData("pager", pager).setTitle(" 登录日志列表"));
return "pages/log/login/list";
}
}
| {'content_hash': 'df9921683263469faf05857387900681', 'timestamp': '', 'source': 'github', 'line_count': 49, 'max_line_length': 129, 'avg_line_length': 34.83673469387755, 'alnum_prop': 0.7551259519625073, 'repo_name': 'Kerbores/spring-thunder', 'id': '5e7f1b6c239cfc9c61ab48b1e68f6e67aa7a7765', 'size': '1731', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'spring-thunder/src/main/java/club/zhcs/thunder/controller/admin/log/LoginLogController.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'CSS', 'bytes': '4136035'}, {'name': 'CoffeeScript', 'bytes': '83631'}, {'name': 'HTML', 'bytes': '1402832'}, {'name': 'Java', 'bytes': '157946'}, {'name': 'JavaScript', 'bytes': '18705408'}, {'name': 'PHP', 'bytes': '12187'}, {'name': 'Shell', 'bytes': '444'}]} |
<?php
class ClassHelper
{
protected $origin;
/** @var ReflectionClass */
protected $target;
/**
* Creates and returns the wrapper.
* Only objects are allowed to be passed, else an exception will be thrown.
* Store the input object to the created wrapper.
*
* @param $object
*
* @return Helper
*/
public static function instance($object)
{
if (!is_object($object)) {
throw new \InvalidArgumentException('Only objects are allowed to be passed to the helper.');
}
$response = new self();
$response->origin = $object;
$response->target = new ReflectionClass($object);
return $response;
}
public function __get($name)
{
$property = $this->getProperty($name);
$value = $property->getValue($this->origin);
return $value;
}
public function __set($name, $value)
{
$property = $this->getProperty($name);
$property->setValue($this->origin, $value);
return true;
}
protected function getProperty($name)
{
if (!$this->target->hasProperty($name)) {
$message = sprintf('Property not found on object %s.', get_class($this->origin));
throw new OutOfBoundsException($message);
}
/** @var ReflectionProperty $property */
$property = $this->target->getProperty($name);
$property->setAccessible(true);
return $property;
}
public function call($name, array $args = [])
{
if (!$this->target->hasMethod($name)) {
$message = sprintf('Method not found on object %s.', get_class($this->origin));
throw new BadMethodCallException($message);
}
$method = $this->target->getMethod($name);
$method->setAccessible(true);
$response = $method->invokeArgs($this->origin, $args);
return $response;
}
}
| {'content_hash': '355cce27bc3a0de459312cc06660848c', 'timestamp': '', 'source': 'github', 'line_count': 71, 'max_line_length': 104, 'avg_line_length': 27.380281690140844, 'alnum_prop': 0.5720164609053497, 'repo_name': 'bogdananton/php-class-helper', 'id': 'cc9796b16242c263b39450bc72da0e6205c12628', 'size': '1944', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'ClassHelper.php', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'PHP', 'bytes': '8106'}]} |
'use strict';
if (process.platform === 'darwin') {
module.exports = require('./lib/osx');
module.exports.sync = require('./lib/osx').sync;
} else if (process.platform === 'win32') {
module.exports = require('./lib/win');
module.exports.sync = require('./lib/win').sync;
} else {
module.exports = require('./lib/linux');
module.exports.sync = require('./lib/linux').sync;
}
| {'content_hash': '118d8353fb769b5443149d540d33898c', 'timestamp': '', 'source': 'github', 'line_count': 12, 'max_line_length': 51, 'avg_line_length': 31.666666666666668, 'alnum_prop': 0.6473684210526316, 'repo_name': 'kevva/wifi-name', 'id': '5052fd83072d797bf2c203ec5708d695982293e2', 'size': '380', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'index.js', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'JavaScript', 'bytes': '2717'}]} |
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<!-- NewPage -->
<html lang="en">
<head>
<!-- Generated by javadoc (version 1.7.0_72) on Wed May 13 11:47:39 EDT 2015 -->
<title>UntypedResultSet.Row (apache-cassandra API)</title>
<meta name="date" content="2015-05-13">
<link rel="stylesheet" type="text/css" href="../../../../stylesheet.css" title="Style">
</head>
<body>
<script type="text/javascript"><!--
if (location.href.indexOf('is-external=true') == -1) {
parent.document.title="UntypedResultSet.Row (apache-cassandra API)";
}
//-->
</script>
<noscript>
<div>JavaScript is disabled on your browser.</div>
</noscript>
<!-- ========= START OF TOP NAVBAR ======= -->
<div class="topNav"><a name="navbar_top">
<!-- -->
</a><a href="#skip-navbar_top" title="Skip navigation links"></a><a name="navbar_top_firstrow">
<!-- -->
</a>
<ul class="navList" title="Navigation">
<li><a href="../../../../overview-summary.html">Overview</a></li>
<li><a href="package-summary.html">Package</a></li>
<li class="navBarCell1Rev">Class</li>
<li><a href="class-use/UntypedResultSet.Row.html">Use</a></li>
<li><a href="package-tree.html">Tree</a></li>
<li><a href="../../../../deprecated-list.html">Deprecated</a></li>
<li><a href="../../../../index-all.html">Index</a></li>
<li><a href="../../../../help-doc.html">Help</a></li>
</ul>
</div>
<div class="subNav">
<ul class="navList">
<li><a href="../../../../org/apache/cassandra/cql3/UntypedResultSet.html" title="class in org.apache.cassandra.cql3"><span class="strong">Prev Class</span></a></li>
<li><a href="../../../../org/apache/cassandra/cql3/UpdateParameters.html" title="class in org.apache.cassandra.cql3"><span class="strong">Next Class</span></a></li>
</ul>
<ul class="navList">
<li><a href="../../../../index.html?org/apache/cassandra/cql3/UntypedResultSet.Row.html" target="_top">Frames</a></li>
<li><a href="UntypedResultSet.Row.html" target="_top">No Frames</a></li>
</ul>
<ul class="navList" id="allclasses_navbar_top">
<li><a href="../../../../allclasses-noframe.html">All Classes</a></li>
</ul>
<div>
<script type="text/javascript"><!--
allClassesLink = document.getElementById("allclasses_navbar_top");
if(window==top) {
allClassesLink.style.display = "block";
}
else {
allClassesLink.style.display = "none";
}
//-->
</script>
</div>
<div>
<ul class="subNavList">
<li>Summary: </li>
<li>Nested | </li>
<li>Field | </li>
<li><a href="#constructor_summary">Constr</a> | </li>
<li><a href="#method_summary">Method</a></li>
</ul>
<ul class="subNavList">
<li>Detail: </li>
<li>Field | </li>
<li><a href="#constructor_detail">Constr</a> | </li>
<li><a href="#method_detail">Method</a></li>
</ul>
</div>
<a name="skip-navbar_top">
<!-- -->
</a></div>
<!-- ========= END OF TOP NAVBAR ========= -->
<!-- ======== START OF CLASS DATA ======== -->
<div class="header">
<div class="subTitle">org.apache.cassandra.cql3</div>
<h2 title="Class UntypedResultSet.Row" class="title">Class UntypedResultSet.Row</h2>
</div>
<div class="contentContainer">
<ul class="inheritance">
<li>java.lang.Object</li>
<li>
<ul class="inheritance">
<li>org.apache.cassandra.cql3.UntypedResultSet.Row</li>
</ul>
</li>
</ul>
<div class="description">
<ul class="blockList">
<li class="blockList">
<dl>
<dt>Enclosing class:</dt>
<dd><a href="../../../../org/apache/cassandra/cql3/UntypedResultSet.html" title="class in org.apache.cassandra.cql3">UntypedResultSet</a></dd>
</dl>
<hr>
<br>
<pre>public static class <span class="strong">UntypedResultSet.Row</span>
extends java.lang.Object</pre>
</li>
</ul>
</div>
<div class="summary">
<ul class="blockList">
<li class="blockList">
<!-- ======== CONSTRUCTOR SUMMARY ======== -->
<ul class="blockList">
<li class="blockList"><a name="constructor_summary">
<!-- -->
</a>
<h3>Constructor Summary</h3>
<table class="overviewSummary" border="0" cellpadding="3" cellspacing="0" summary="Constructor Summary table, listing constructors, and an explanation">
<caption><span>Constructors</span><span class="tabEnd"> </span></caption>
<tr>
<th class="colOne" scope="col">Constructor and Description</th>
</tr>
<tr class="altColor">
<td class="colOne"><code><strong><a href="../../../../org/apache/cassandra/cql3/UntypedResultSet.Row.html#UntypedResultSet.Row(java.util.List,%20java.util.List)">UntypedResultSet.Row</a></strong>(java.util.List<<a href="../../../../org/apache/cassandra/cql3/ColumnSpecification.html" title="class in org.apache.cassandra.cql3">ColumnSpecification</a>> names,
java.util.List<java.nio.ByteBuffer> columns)</code> </td>
</tr>
<tr class="rowColor">
<td class="colOne"><code><strong><a href="../../../../org/apache/cassandra/cql3/UntypedResultSet.Row.html#UntypedResultSet.Row(java.util.Map)">UntypedResultSet.Row</a></strong>(java.util.Map<java.lang.String,java.nio.ByteBuffer> data)</code> </td>
</tr>
</table>
</li>
</ul>
<!-- ========== METHOD SUMMARY =========== -->
<ul class="blockList">
<li class="blockList"><a name="method_summary">
<!-- -->
</a>
<h3>Method Summary</h3>
<table class="overviewSummary" border="0" cellpadding="3" cellspacing="0" summary="Method Summary table, listing methods, and an explanation">
<caption><span>Methods</span><span class="tabEnd"> </span></caption>
<tr>
<th class="colFirst" scope="col">Modifier and Type</th>
<th class="colLast" scope="col">Method and Description</th>
</tr>
<tr class="altColor">
<td class="colFirst"><code>boolean</code></td>
<td class="colLast"><code><strong><a href="../../../../org/apache/cassandra/cql3/UntypedResultSet.Row.html#getBoolean(java.lang.String)">getBoolean</a></strong>(java.lang.String column)</code> </td>
</tr>
<tr class="rowColor">
<td class="colFirst"><code>java.nio.ByteBuffer</code></td>
<td class="colLast"><code><strong><a href="../../../../org/apache/cassandra/cql3/UntypedResultSet.Row.html#getBytes(java.lang.String)">getBytes</a></strong>(java.lang.String column)</code> </td>
</tr>
<tr class="altColor">
<td class="colFirst"><code>java.util.List<<a href="../../../../org/apache/cassandra/cql3/ColumnSpecification.html" title="class in org.apache.cassandra.cql3">ColumnSpecification</a>></code></td>
<td class="colLast"><code><strong><a href="../../../../org/apache/cassandra/cql3/UntypedResultSet.Row.html#getColumns()">getColumns</a></strong>()</code> </td>
</tr>
<tr class="rowColor">
<td class="colFirst"><code>double</code></td>
<td class="colLast"><code><strong><a href="../../../../org/apache/cassandra/cql3/UntypedResultSet.Row.html#getDouble(java.lang.String)">getDouble</a></strong>(java.lang.String column)</code> </td>
</tr>
<tr class="altColor">
<td class="colFirst"><code>java.net.InetAddress</code></td>
<td class="colLast"><code><strong><a href="../../../../org/apache/cassandra/cql3/UntypedResultSet.Row.html#getInetAddress(java.lang.String)">getInetAddress</a></strong>(java.lang.String column)</code> </td>
</tr>
<tr class="rowColor">
<td class="colFirst"><code>int</code></td>
<td class="colLast"><code><strong><a href="../../../../org/apache/cassandra/cql3/UntypedResultSet.Row.html#getInt(java.lang.String)">getInt</a></strong>(java.lang.String column)</code> </td>
</tr>
<tr class="altColor">
<td class="colFirst"><code><T> java.util.List<T></code></td>
<td class="colLast"><code><strong><a href="../../../../org/apache/cassandra/cql3/UntypedResultSet.Row.html#getList(java.lang.String,%20org.apache.cassandra.db.marshal.AbstractType)">getList</a></strong>(java.lang.String column,
<a href="../../../../org/apache/cassandra/db/marshal/AbstractType.html" title="class in org.apache.cassandra.db.marshal">AbstractType</a><T> type)</code> </td>
</tr>
<tr class="rowColor">
<td class="colFirst"><code>long</code></td>
<td class="colLast"><code><strong><a href="../../../../org/apache/cassandra/cql3/UntypedResultSet.Row.html#getLong(java.lang.String)">getLong</a></strong>(java.lang.String column)</code> </td>
</tr>
<tr class="altColor">
<td class="colFirst"><code><K,V> java.util.Map<K,V></code></td>
<td class="colLast"><code><strong><a href="../../../../org/apache/cassandra/cql3/UntypedResultSet.Row.html#getMap(java.lang.String,%20org.apache.cassandra.db.marshal.AbstractType,%20org.apache.cassandra.db.marshal.AbstractType)">getMap</a></strong>(java.lang.String column,
<a href="../../../../org/apache/cassandra/db/marshal/AbstractType.html" title="class in org.apache.cassandra.db.marshal">AbstractType</a><K> keyType,
<a href="../../../../org/apache/cassandra/db/marshal/AbstractType.html" title="class in org.apache.cassandra.db.marshal">AbstractType</a><V> valueType)</code> </td>
</tr>
<tr class="rowColor">
<td class="colFirst"><code><T> java.util.Set<T></code></td>
<td class="colLast"><code><strong><a href="../../../../org/apache/cassandra/cql3/UntypedResultSet.Row.html#getSet(java.lang.String,%20org.apache.cassandra.db.marshal.AbstractType)">getSet</a></strong>(java.lang.String column,
<a href="../../../../org/apache/cassandra/db/marshal/AbstractType.html" title="class in org.apache.cassandra.db.marshal">AbstractType</a><T> type)</code> </td>
</tr>
<tr class="altColor">
<td class="colFirst"><code>java.lang.String</code></td>
<td class="colLast"><code><strong><a href="../../../../org/apache/cassandra/cql3/UntypedResultSet.Row.html#getString(java.lang.String)">getString</a></strong>(java.lang.String column)</code> </td>
</tr>
<tr class="rowColor">
<td class="colFirst"><code>java.util.Date</code></td>
<td class="colLast"><code><strong><a href="../../../../org/apache/cassandra/cql3/UntypedResultSet.Row.html#getTimestamp(java.lang.String)">getTimestamp</a></strong>(java.lang.String column)</code> </td>
</tr>
<tr class="altColor">
<td class="colFirst"><code>java.util.UUID</code></td>
<td class="colLast"><code><strong><a href="../../../../org/apache/cassandra/cql3/UntypedResultSet.Row.html#getUUID(java.lang.String)">getUUID</a></strong>(java.lang.String column)</code> </td>
</tr>
<tr class="rowColor">
<td class="colFirst"><code>boolean</code></td>
<td class="colLast"><code><strong><a href="../../../../org/apache/cassandra/cql3/UntypedResultSet.Row.html#has(java.lang.String)">has</a></strong>(java.lang.String column)</code> </td>
</tr>
<tr class="altColor">
<td class="colFirst"><code>java.lang.String</code></td>
<td class="colLast"><code><strong><a href="../../../../org/apache/cassandra/cql3/UntypedResultSet.Row.html#toString()">toString</a></strong>()</code> </td>
</tr>
</table>
<ul class="blockList">
<li class="blockList"><a name="methods_inherited_from_class_java.lang.Object">
<!-- -->
</a>
<h3>Methods inherited from class java.lang.Object</h3>
<code>clone, equals, finalize, getClass, hashCode, notify, notifyAll, wait, wait, wait</code></li>
</ul>
</li>
</ul>
</li>
</ul>
</div>
<div class="details">
<ul class="blockList">
<li class="blockList">
<!-- ========= CONSTRUCTOR DETAIL ======== -->
<ul class="blockList">
<li class="blockList"><a name="constructor_detail">
<!-- -->
</a>
<h3>Constructor Detail</h3>
<a name="UntypedResultSet.Row(java.util.Map)">
<!-- -->
</a>
<ul class="blockList">
<li class="blockList">
<h4>UntypedResultSet.Row</h4>
<pre>public UntypedResultSet.Row(java.util.Map<java.lang.String,java.nio.ByteBuffer> data)</pre>
</li>
</ul>
<a name="UntypedResultSet.Row(java.util.List, java.util.List)">
<!-- -->
</a>
<ul class="blockListLast">
<li class="blockList">
<h4>UntypedResultSet.Row</h4>
<pre>public UntypedResultSet.Row(java.util.List<<a href="../../../../org/apache/cassandra/cql3/ColumnSpecification.html" title="class in org.apache.cassandra.cql3">ColumnSpecification</a>> names,
java.util.List<java.nio.ByteBuffer> columns)</pre>
</li>
</ul>
</li>
</ul>
<!-- ============ METHOD DETAIL ========== -->
<ul class="blockList">
<li class="blockList"><a name="method_detail">
<!-- -->
</a>
<h3>Method Detail</h3>
<a name="has(java.lang.String)">
<!-- -->
</a>
<ul class="blockList">
<li class="blockList">
<h4>has</h4>
<pre>public boolean has(java.lang.String column)</pre>
</li>
</ul>
<a name="getString(java.lang.String)">
<!-- -->
</a>
<ul class="blockList">
<li class="blockList">
<h4>getString</h4>
<pre>public java.lang.String getString(java.lang.String column)</pre>
</li>
</ul>
<a name="getBoolean(java.lang.String)">
<!-- -->
</a>
<ul class="blockList">
<li class="blockList">
<h4>getBoolean</h4>
<pre>public boolean getBoolean(java.lang.String column)</pre>
</li>
</ul>
<a name="getInt(java.lang.String)">
<!-- -->
</a>
<ul class="blockList">
<li class="blockList">
<h4>getInt</h4>
<pre>public int getInt(java.lang.String column)</pre>
</li>
</ul>
<a name="getDouble(java.lang.String)">
<!-- -->
</a>
<ul class="blockList">
<li class="blockList">
<h4>getDouble</h4>
<pre>public double getDouble(java.lang.String column)</pre>
</li>
</ul>
<a name="getBytes(java.lang.String)">
<!-- -->
</a>
<ul class="blockList">
<li class="blockList">
<h4>getBytes</h4>
<pre>public java.nio.ByteBuffer getBytes(java.lang.String column)</pre>
</li>
</ul>
<a name="getInetAddress(java.lang.String)">
<!-- -->
</a>
<ul class="blockList">
<li class="blockList">
<h4>getInetAddress</h4>
<pre>public java.net.InetAddress getInetAddress(java.lang.String column)</pre>
</li>
</ul>
<a name="getUUID(java.lang.String)">
<!-- -->
</a>
<ul class="blockList">
<li class="blockList">
<h4>getUUID</h4>
<pre>public java.util.UUID getUUID(java.lang.String column)</pre>
</li>
</ul>
<a name="getTimestamp(java.lang.String)">
<!-- -->
</a>
<ul class="blockList">
<li class="blockList">
<h4>getTimestamp</h4>
<pre>public java.util.Date getTimestamp(java.lang.String column)</pre>
</li>
</ul>
<a name="getLong(java.lang.String)">
<!-- -->
</a>
<ul class="blockList">
<li class="blockList">
<h4>getLong</h4>
<pre>public long getLong(java.lang.String column)</pre>
</li>
</ul>
<a name="getSet(java.lang.String, org.apache.cassandra.db.marshal.AbstractType)">
<!-- -->
</a>
<ul class="blockList">
<li class="blockList">
<h4>getSet</h4>
<pre>public <T> java.util.Set<T> getSet(java.lang.String column,
<a href="../../../../org/apache/cassandra/db/marshal/AbstractType.html" title="class in org.apache.cassandra.db.marshal">AbstractType</a><T> type)</pre>
</li>
</ul>
<a name="getList(java.lang.String, org.apache.cassandra.db.marshal.AbstractType)">
<!-- -->
</a>
<ul class="blockList">
<li class="blockList">
<h4>getList</h4>
<pre>public <T> java.util.List<T> getList(java.lang.String column,
<a href="../../../../org/apache/cassandra/db/marshal/AbstractType.html" title="class in org.apache.cassandra.db.marshal">AbstractType</a><T> type)</pre>
</li>
</ul>
<a name="getMap(java.lang.String, org.apache.cassandra.db.marshal.AbstractType, org.apache.cassandra.db.marshal.AbstractType)">
<!-- -->
</a>
<ul class="blockList">
<li class="blockList">
<h4>getMap</h4>
<pre>public <K,V> java.util.Map<K,V> getMap(java.lang.String column,
<a href="../../../../org/apache/cassandra/db/marshal/AbstractType.html" title="class in org.apache.cassandra.db.marshal">AbstractType</a><K> keyType,
<a href="../../../../org/apache/cassandra/db/marshal/AbstractType.html" title="class in org.apache.cassandra.db.marshal">AbstractType</a><V> valueType)</pre>
</li>
</ul>
<a name="getColumns()">
<!-- -->
</a>
<ul class="blockList">
<li class="blockList">
<h4>getColumns</h4>
<pre>public java.util.List<<a href="../../../../org/apache/cassandra/cql3/ColumnSpecification.html" title="class in org.apache.cassandra.cql3">ColumnSpecification</a>> getColumns()</pre>
</li>
</ul>
<a name="toString()">
<!-- -->
</a>
<ul class="blockListLast">
<li class="blockList">
<h4>toString</h4>
<pre>public java.lang.String toString()</pre>
<dl>
<dt><strong>Overrides:</strong></dt>
<dd><code>toString</code> in class <code>java.lang.Object</code></dd>
</dl>
</li>
</ul>
</li>
</ul>
</li>
</ul>
</div>
</div>
<!-- ========= END OF CLASS DATA ========= -->
<!-- ======= START OF BOTTOM NAVBAR ====== -->
<div class="bottomNav"><a name="navbar_bottom">
<!-- -->
</a><a href="#skip-navbar_bottom" title="Skip navigation links"></a><a name="navbar_bottom_firstrow">
<!-- -->
</a>
<ul class="navList" title="Navigation">
<li><a href="../../../../overview-summary.html">Overview</a></li>
<li><a href="package-summary.html">Package</a></li>
<li class="navBarCell1Rev">Class</li>
<li><a href="class-use/UntypedResultSet.Row.html">Use</a></li>
<li><a href="package-tree.html">Tree</a></li>
<li><a href="../../../../deprecated-list.html">Deprecated</a></li>
<li><a href="../../../../index-all.html">Index</a></li>
<li><a href="../../../../help-doc.html">Help</a></li>
</ul>
</div>
<div class="subNav">
<ul class="navList">
<li><a href="../../../../org/apache/cassandra/cql3/UntypedResultSet.html" title="class in org.apache.cassandra.cql3"><span class="strong">Prev Class</span></a></li>
<li><a href="../../../../org/apache/cassandra/cql3/UpdateParameters.html" title="class in org.apache.cassandra.cql3"><span class="strong">Next Class</span></a></li>
</ul>
<ul class="navList">
<li><a href="../../../../index.html?org/apache/cassandra/cql3/UntypedResultSet.Row.html" target="_top">Frames</a></li>
<li><a href="UntypedResultSet.Row.html" target="_top">No Frames</a></li>
</ul>
<ul class="navList" id="allclasses_navbar_bottom">
<li><a href="../../../../allclasses-noframe.html">All Classes</a></li>
</ul>
<div>
<script type="text/javascript"><!--
allClassesLink = document.getElementById("allclasses_navbar_bottom");
if(window==top) {
allClassesLink.style.display = "block";
}
else {
allClassesLink.style.display = "none";
}
//-->
</script>
</div>
<div>
<ul class="subNavList">
<li>Summary: </li>
<li>Nested | </li>
<li>Field | </li>
<li><a href="#constructor_summary">Constr</a> | </li>
<li><a href="#method_summary">Method</a></li>
</ul>
<ul class="subNavList">
<li>Detail: </li>
<li>Field | </li>
<li><a href="#constructor_detail">Constr</a> | </li>
<li><a href="#method_detail">Method</a></li>
</ul>
</div>
<a name="skip-navbar_bottom">
<!-- -->
</a></div>
<!-- ======== END OF BOTTOM NAVBAR ======= -->
<p class="legalCopy"><small>Copyright © 2015 The Apache Software Foundation</small></p>
</body>
</html>
| {'content_hash': 'ddfb199bf005eb9a6ee375accbe38991', 'timestamp': '', 'source': 'github', 'line_count': 469, 'max_line_length': 369, 'avg_line_length': 40.61620469083156, 'alnum_prop': 0.660979578980524, 'repo_name': 'anuragkapur/cassandra-2.1.2-ak-skynet', 'id': '8975ec467a0c2750dfff936bb29ea8033dbbcde8', 'size': '19049', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'apache-cassandra-2.0.15/javadoc/org/apache/cassandra/cql3/UntypedResultSet.Row.html', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Batchfile', 'bytes': '59670'}, {'name': 'PowerShell', 'bytes': '37758'}, {'name': 'Python', 'bytes': '622552'}, {'name': 'Shell', 'bytes': '100474'}, {'name': 'Thrift', 'bytes': '78926'}]} |
package com.orientechnologies.orient.server.distributed;
import static org.junit.Assert.assertEquals;
import static org.junit.Assert.assertTrue;
import com.orientechnologies.orient.core.config.OGlobalConfiguration;
import com.orientechnologies.orient.core.db.ODatabaseSession;
import com.orientechnologies.orient.core.db.OrientDB;
import com.orientechnologies.orient.core.db.OrientDBConfig;
import com.orientechnologies.orient.core.db.document.ODatabaseDocumentTx;
import com.orientechnologies.orient.core.metadata.schema.OClass;
import com.orientechnologies.orient.core.record.OVertex;
import com.orientechnologies.orient.core.sql.executor.OResult;
import com.orientechnologies.orient.core.sql.executor.OResultSet;
import com.orientechnologies.orient.setup.SetupConfig;
import com.orientechnologies.orient.setup.TestSetup;
import com.orientechnologies.orient.setup.TestSetupUtil;
import com.orientechnologies.orient.setup.configs.SimpleDServerConfig;
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
public class SimpleQueryDistributedIT {
private TestSetup setup;
private SetupConfig config;
private String server0, server1, server2;
private OrientDB remote;
private ODatabaseSession session;
@Before
public void before() throws Exception {
config = new SimpleDServerConfig();
server0 = SimpleDServerConfig.SERVER0;
server1 = SimpleDServerConfig.SERVER1;
server2 = SimpleDServerConfig.SERVER2;
setup = TestSetupUtil.create(config);
setup.setup();
remote = setup.createRemote(server0, "root", "test", OrientDBConfig.defaultConfig());
remote.execute(
"create database ? plocal users(admin identified by 'admin' role admin)", "test");
session = remote.open("test", "admin", "admin");
}
@Test
public void test() {
OVertex vertex = session.newVertex("V");
vertex.setProperty("name", "one");
session.save(vertex);
// Query with SQL
OResultSet res = session.query("select from V");
assertTrue(res.hasNext());
assertEquals(res.next().getProperty("name"), "one");
// Query with script
res = session.execute("sql", "select from V");
assertTrue(res.hasNext());
assertEquals(res.next().getProperty("name"), "one");
// Query order by
OClass v2 = session.createVertexClass("V2");
int records = (OGlobalConfiguration.QUERY_REMOTE_RESULTSET_PAGE_SIZE.getValueAsInteger() + 10);
for (int i = 0; i < records; i++) {
vertex = session.newVertex("V2");
vertex.setProperty("name", "one");
vertex.setProperty("pos", i);
session.save(vertex);
}
res = session.query("select from V2 order by pos");
for (int i = 0; i < records; i++) {
assertTrue(res.hasNext());
OResult ele = res.next();
assertEquals((int) ele.getProperty("pos"), i);
assertEquals(ele.getProperty("name"), "one");
}
}
@After
public void after() throws InterruptedException {
System.out.println("Tearing down test setup.");
try {
if (remote != null) {
remote.drop("test");
remote.close();
}
} finally {
setup.teardown();
ODatabaseDocumentTx.closeAll();
}
}
}
| {'content_hash': 'cb9f578fcbbed6ae08f8256a46d1f433', 'timestamp': '', 'source': 'github', 'line_count': 95, 'max_line_length': 99, 'avg_line_length': 33.56842105263158, 'alnum_prop': 0.7118218877391032, 'repo_name': 'orientechnologies/orientdb', 'id': 'b700fbd44b868ed811e4a17f226b1c64c41391fc', 'size': '3189', 'binary': False, 'copies': '1', 'ref': 'refs/heads/develop', 'path': 'distributed/src/test/java/com/orientechnologies/orient/server/distributed/SimpleQueryDistributedIT.java', 'mode': '33261', 'license': 'apache-2.0', 'language': [{'name': 'Batchfile', 'bytes': '19302'}, {'name': 'Dockerfile', 'bytes': '705'}, {'name': 'Gnuplot', 'bytes': '1245'}, {'name': 'Groovy', 'bytes': '7913'}, {'name': 'HTML', 'bytes': '5750'}, {'name': 'Java', 'bytes': '26588383'}, {'name': 'JavaScript', 'bytes': '259'}, {'name': 'PLpgSQL', 'bytes': '54881'}, {'name': 'Shell', 'bytes': '33650'}]} |
package org.thymeleaf.spring6.processor;
import org.springframework.util.StringUtils;
import org.thymeleaf.context.ITemplateContext;
import org.thymeleaf.engine.AttributeDefinition;
import org.thymeleaf.engine.AttributeDefinitions;
import org.thymeleaf.engine.AttributeName;
import org.thymeleaf.engine.IAttributeDefinitionsAware;
import org.thymeleaf.exceptions.TemplateProcessingException;
import org.thymeleaf.model.IProcessableElementTag;
import org.thymeleaf.processor.element.AbstractAttributeTagProcessor;
import org.thymeleaf.processor.element.IElementTagStructureHandler;
import org.thymeleaf.spring6.context.IThymeleafBindStatus;
import org.thymeleaf.spring6.naming.SpringContextVariableNames;
import org.thymeleaf.spring6.util.FieldUtils;
import org.thymeleaf.templatemode.TemplateMode;
import org.thymeleaf.util.Validate;
/**
* Binds an input property with the value in the form's backing bean.
* <p>
* Values for {@code th:field} attributes must be selection expressions
* {@code (*{...})}, as they will be evaluated on the form backing bean and not
* on the context variables (model attributes in Spring MVC jargon).
*
* @author Daniel Fernández
* @since 3.0.3
*/
public abstract class AbstractSpringFieldTagProcessor
extends AbstractAttributeTagProcessor
implements IAttributeDefinitionsAware {
public static final int ATTR_PRECEDENCE = 1700;
public static final String ATTR_NAME = "field";
private static final TemplateMode TEMPLATE_MODE = TemplateMode.HTML;
protected static final String INPUT_TAG_NAME = "input";
protected static final String SELECT_TAG_NAME = "select";
protected static final String OPTION_TAG_NAME = "option";
protected static final String TEXTAREA_TAG_NAME = "textarea";
protected static final String ID_ATTR_NAME = "id";
protected static final String TYPE_ATTR_NAME = "type";
protected static final String NAME_ATTR_NAME = "name";
protected static final String VALUE_ATTR_NAME = "value";
protected static final String CHECKED_ATTR_NAME = "checked";
protected static final String SELECTED_ATTR_NAME = "selected";
protected static final String DISABLED_ATTR_NAME = "disabled";
protected static final String MULTIPLE_ATTR_NAME = "multiple";
private AttributeDefinition discriminatorAttributeDefinition;
protected AttributeDefinition idAttributeDefinition;
protected AttributeDefinition typeAttributeDefinition;
protected AttributeDefinition nameAttributeDefinition;
protected AttributeDefinition valueAttributeDefinition;
protected AttributeDefinition checkedAttributeDefinition;
protected AttributeDefinition selectedAttributeDefinition;
protected AttributeDefinition disabledAttributeDefinition;
protected AttributeDefinition multipleAttributeDefinition;
private final String discriminatorAttrName;
private final String[] discriminatorAttrValues;
private final boolean removeAttribute;
public AbstractSpringFieldTagProcessor(
final String dialectPrefix, final String elementName,
final String discriminatorAttrName, final String[] discriminatorAttrValues,
final boolean removeAttribute) {
super(TEMPLATE_MODE, dialectPrefix, elementName, false, ATTR_NAME, true, ATTR_PRECEDENCE, false);
this.discriminatorAttrName = discriminatorAttrName;
this.discriminatorAttrValues = discriminatorAttrValues;
this.removeAttribute = removeAttribute;
}
public void setAttributeDefinitions(final AttributeDefinitions attributeDefinitions) {
Validate.notNull(attributeDefinitions, "Attribute Definitions cannot be null");
// We precompute the AttributeDefinitions in order to being able to use much
// faster methods for setting/replacing attributes on the ElementAttributes implementation
this.discriminatorAttributeDefinition =
(this.discriminatorAttrName != null? attributeDefinitions.forName(TEMPLATE_MODE, this.discriminatorAttrName) : null);
this.idAttributeDefinition = attributeDefinitions.forName(TEMPLATE_MODE, ID_ATTR_NAME);
this.typeAttributeDefinition = attributeDefinitions.forName(TEMPLATE_MODE, TYPE_ATTR_NAME);
this.nameAttributeDefinition = attributeDefinitions.forName(TEMPLATE_MODE, NAME_ATTR_NAME);
this.valueAttributeDefinition = attributeDefinitions.forName(TEMPLATE_MODE, VALUE_ATTR_NAME);
this.checkedAttributeDefinition = attributeDefinitions.forName(TEMPLATE_MODE, CHECKED_ATTR_NAME);
this.selectedAttributeDefinition = attributeDefinitions.forName(TEMPLATE_MODE, SELECTED_ATTR_NAME);
this.disabledAttributeDefinition = attributeDefinitions.forName(TEMPLATE_MODE, DISABLED_ATTR_NAME);
this.multipleAttributeDefinition = attributeDefinitions.forName(TEMPLATE_MODE, MULTIPLE_ATTR_NAME);
}
private boolean matchesDiscriminator(final IProcessableElementTag tag) {
if (this.discriminatorAttrName == null) {
return true;
}
final boolean hasDiscriminatorAttr = tag.hasAttribute(this.discriminatorAttributeDefinition.getAttributeName());
if (this.discriminatorAttrValues == null || this.discriminatorAttrValues.length == 0) {
return hasDiscriminatorAttr;
}
final String discriminatorTagValue =
(hasDiscriminatorAttr? tag.getAttributeValue(this.discriminatorAttributeDefinition.getAttributeName()) : null);
for (int i = 0; i < this.discriminatorAttrValues.length; i++) {
final String discriminatorAttrValue = this.discriminatorAttrValues[i];
if (discriminatorAttrValue == null) {
if (!hasDiscriminatorAttr || discriminatorTagValue == null) {
return true;
}
} else if (discriminatorAttrValue.equals(discriminatorTagValue)) {
return true;
}
}
return false;
}
@Override
protected void doProcess(
final ITemplateContext context,
final IProcessableElementTag tag,
final AttributeName attributeName, final String attributeValue,
final IElementTagStructureHandler structureHandler) {
/*
* First thing to check is whether this processor really matches, because so far we have asked the engine only
* to match per attribute (th:field) and host tag (input, select, option...) but we still don't know if the
* match is complete because we might still need to assess for example that the 'type' attribute has the
* correct value. For example, the same processor will not be executing on <input type="text" th:field="*{a}"/>
* and on <input type="checkbox" th:field="*{a}"/>
*/
if (!matchesDiscriminator(tag)) {
// Note in this case we do not have to remove the th:field attribute because the correct processor is still
// to be executed!
return;
}
if (this.removeAttribute) {
structureHandler.removeAttribute(attributeName);
}
final IThymeleafBindStatus bindStatus = FieldUtils.getBindStatus(context, attributeValue);
if (bindStatus == null) {
throw new TemplateProcessingException(
"Cannot process attribute '" + attributeName + "': no associated BindStatus could be found for " +
"the intended form binding operations. This can be due to the lack of a proper management of the " +
"Spring RequestContext, which is usually done through the ThymeleafView or ThymeleafReactiveView");
}
// We set the BindStatus into a local variable just in case we have more BindStatus-related processors to
// be applied for the same tag, like for example a th:errorclass
structureHandler.setLocalVariable(SpringContextVariableNames.THYMELEAF_FIELD_BIND_STATUS, bindStatus);
doProcess(context, tag, attributeName, attributeValue, bindStatus, structureHandler);
}
protected abstract void doProcess(
final ITemplateContext context,
final IProcessableElementTag tag,
final AttributeName attributeName,
final String attributeValue,
final IThymeleafBindStatus bindStatus,
final IElementTagStructureHandler structureHandler);
// This method is designed to be called from the diverse subclasses
protected final String computeId(
final ITemplateContext context,
final IProcessableElementTag tag,
final String name, final boolean sequence) {
String id = tag.getAttributeValue(this.idAttributeDefinition.getAttributeName());
if (!org.thymeleaf.util.StringUtils.isEmptyOrWhitespace(id)) {
return (StringUtils.hasText(id) ? id : null);
}
id = FieldUtils.idFromName(name);
if (sequence) {
final Integer count = context.getIdentifierSequences().getAndIncrementIDSeq(id);
return id + count.toString();
}
return id;
}
}
| {'content_hash': 'e583ddbb00545fad6c919b83b65d762a', 'timestamp': '', 'source': 'github', 'line_count': 211, 'max_line_length': 133, 'avg_line_length': 43.5260663507109, 'alnum_prop': 0.7192944250871081, 'repo_name': 'thymeleaf/thymeleaf-spring', 'id': '8ab55cd158f10b42c25bcdbda2c2cd664f5cc721', 'size': '10010', 'binary': False, 'copies': '2', 'ref': 'refs/heads/3.1-master', 'path': 'thymeleaf-spring6/src/main/java/org/thymeleaf/spring6/processor/AbstractSpringFieldTagProcessor.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Java', 'bytes': '1170794'}]} |
package org.apache.zookeeper.server;
import java.io.BufferedWriter;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.PrintWriter;
import java.io.Writer;
import java.net.InetAddress;
import java.net.InetSocketAddress;
import java.nio.ByteBuffer;
import java.nio.channels.CancelledKeyException;
import java.nio.channels.SelectionKey;
import java.nio.channels.SocketChannel;
import java.security.cert.Certificate;
import java.util.Queue;
import java.util.concurrent.LinkedBlockingQueue;
import java.util.concurrent.atomic.AtomicBoolean;
import java.util.concurrent.atomic.AtomicInteger;
import org.apache.jute.BinaryInputArchive;
import org.apache.jute.BinaryOutputArchive;
import org.apache.jute.Record;
import org.apache.zookeeper.WatchedEvent;
import org.apache.zookeeper.data.Id;
import org.apache.zookeeper.proto.ReplyHeader;
import org.apache.zookeeper.proto.RequestHeader;
import org.apache.zookeeper.proto.WatcherEvent;
import org.apache.zookeeper.server.NIOServerCnxnFactory.SelectorThread;
import org.apache.zookeeper.server.command.CommandExecutor;
import org.apache.zookeeper.server.command.FourLetterCommands;
import org.apache.zookeeper.server.command.SetTraceMaskCommand;
import org.apache.zookeeper.server.command.NopCommand;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* This class handles communication with clients using NIO. There is one per
* client, but only one thread doing the communication.
*/
public class NIOServerCnxn extends ServerCnxn {
private static final Logger LOG = LoggerFactory.getLogger(NIOServerCnxn.class);
private final NIOServerCnxnFactory factory;
private final SocketChannel sock;
private final SelectorThread selectorThread;
private final SelectionKey sk;
private boolean initialized;
private final ByteBuffer lenBuffer = ByteBuffer.allocate(4);
private ByteBuffer incomingBuffer = lenBuffer;
private final Queue<ByteBuffer> outgoingBuffers =
new LinkedBlockingQueue<ByteBuffer>();
private int sessionTimeout;
private final ZooKeeperServer zkServer;
/**
* The number of requests that have been submitted but not yet responded to.
*/
private final AtomicInteger outstandingRequests = new AtomicInteger(0);
/**
* This is the id that uniquely identifies the session of a client. Once
* this session is no longer active, the ephemeral nodes will go away.
*/
private long sessionId;
private final int outstandingLimit;
public NIOServerCnxn(ZooKeeperServer zk, SocketChannel sock,
SelectionKey sk, NIOServerCnxnFactory factory,
SelectorThread selectorThread) throws IOException {
this.zkServer = zk;
this.sock = sock;
this.sk = sk;
this.factory = factory;
this.selectorThread = selectorThread;
if (this.factory.login != null) {
this.zooKeeperSaslServer = new ZooKeeperSaslServer(factory.login);
}
if (zk != null) {
outstandingLimit = zk.getGlobalOutstandingLimit();
} else {
outstandingLimit = 1;
}
sock.socket().setTcpNoDelay(true);
/* set socket linger to false, so that socket close does not block */
sock.socket().setSoLinger(false, -1);
InetAddress addr = ((InetSocketAddress) sock.socket()
.getRemoteSocketAddress()).getAddress();
addAuthInfo(new Id("ip", addr.getHostAddress()));
this.sessionTimeout = factory.sessionlessCnxnTimeout;
}
/* Send close connection packet to the client, doIO will eventually
* close the underlying machinery (like socket, selectorkey, etc...)
*/
public void sendCloseSession() {
sendBuffer(ServerCnxnFactory.closeConn);
}
/**
* send buffer without using the asynchronous
* calls to selector and then close the socket
* @param bb
*/
void sendBufferSync(ByteBuffer bb) {
try {
/* configure socket to be blocking
* so that we dont have to do write in
* a tight while loop
*/
if (bb != ServerCnxnFactory.closeConn) {
if (sock.isOpen()) {
sock.configureBlocking(true);
sock.write(bb);
}
packetSent();
}
} catch (IOException ie) {
LOG.error("Error sending data synchronously ", ie);
}
}
/**
* sendBuffer pushes a byte buffer onto the outgoing buffer queue for
* asynchronous writes.
*/
public void sendBuffer(ByteBuffer bb) {
if (LOG.isTraceEnabled()) {
LOG.trace("Add a buffer to outgoingBuffers, sk " + sk
+ " is valid: " + sk.isValid());
}
outgoingBuffers.add(bb);
requestInterestOpsUpdate();
}
/** Read the request payload (everything following the length prefix) */
private void readPayload() throws IOException, InterruptedException {
if (incomingBuffer.remaining() != 0) { // have we read length bytes?
int rc = sock.read(incomingBuffer); // sock is non-blocking, so ok
if (rc < 0) {
throw new EndOfStreamException(
"Unable to read additional data from client sessionid 0x"
+ Long.toHexString(sessionId)
+ ", likely client has closed socket");
}
}
if (incomingBuffer.remaining() == 0) { // have we read length bytes?
packetReceived();
incomingBuffer.flip();
if (!initialized) {
readConnectRequest();
} else {
readRequest();
}
lenBuffer.clear();
incomingBuffer = lenBuffer;
}
}
/**
* This boolean tracks whether the connection is ready for selection or
* not. A connection is marked as not ready for selection while it is
* processing an IO request. The flag is used to gatekeep pushing interest
* op updates onto the selector.
*/
private final AtomicBoolean selectable = new AtomicBoolean(true);
public boolean isSelectable() {
return sk.isValid() && selectable.get();
}
public void disableSelectable() {
selectable.set(false);
}
public void enableSelectable() {
selectable.set(true);
}
private void requestInterestOpsUpdate() {
if (isSelectable()) {
selectorThread.addInterestOpsUpdateRequest(sk);
}
}
void handleWrite(SelectionKey k) throws IOException, CloseRequestException {
if (outgoingBuffers.isEmpty()) {
return;
}
/*
* This is going to reset the buffer position to 0 and the
* limit to the size of the buffer, so that we can fill it
* with data from the non-direct buffers that we need to
* send.
*/
ByteBuffer directBuffer = NIOServerCnxnFactory.getDirectBuffer();
if (directBuffer == null) {
ByteBuffer[] bufferList = new ByteBuffer[outgoingBuffers.size()];
// Use gathered write call. This updates the positions of the
// byte buffers to reflect the bytes that were written out.
sock.write(outgoingBuffers.toArray(bufferList));
// Remove the buffers that we have sent
ByteBuffer bb;
while ((bb = outgoingBuffers.peek()) != null) {
if (bb == ServerCnxnFactory.closeConn) {
throw new CloseRequestException("close requested");
}
if (bb.remaining() > 0) {
break;
}
packetSent();
outgoingBuffers.remove();
}
} else {
directBuffer.clear();
for (ByteBuffer b : outgoingBuffers) {
if (directBuffer.remaining() < b.remaining()) {
/*
* When we call put later, if the directBuffer is to
* small to hold everything, nothing will be copied,
* so we've got to slice the buffer if it's too big.
*/
b = (ByteBuffer) b.slice().limit(
directBuffer.remaining());
}
/*
* put() is going to modify the positions of both
* buffers, put we don't want to change the position of
* the source buffers (we'll do that after the send, if
* needed), so we save and reset the position after the
* copy
*/
int p = b.position();
directBuffer.put(b);
b.position(p);
if (directBuffer.remaining() == 0) {
break;
}
}
/*
* Do the flip: limit becomes position, position gets set to
* 0. This sets us up for the write.
*/
directBuffer.flip();
int sent = sock.write(directBuffer);
ByteBuffer bb;
// Remove the buffers that we have sent
while ((bb = outgoingBuffers.peek()) != null) {
if (bb == ServerCnxnFactory.closeConn) {
throw new CloseRequestException("close requested");
}
if (sent < bb.remaining()) {
/*
* We only partially sent this buffer, so we update
* the position and exit the loop.
*/
bb.position(bb.position() + sent);
break;
}
packetSent();
/* We've sent the whole buffer, so drop the buffer */
sent -= bb.remaining();
outgoingBuffers.remove();
}
}
}
/**
* Only used in order to allow testing
*/
protected boolean isSocketOpen() {
return sock.isOpen();
}
/**
* Handles read/write IO on connection.
*/
void doIO(SelectionKey k) throws InterruptedException {
try {
if (isSocketOpen() == false) {
LOG.warn("trying to do i/o on a null socket for session:0x"
+ Long.toHexString(sessionId));
return;
}
if (k.isReadable()) {
int rc = sock.read(incomingBuffer);
if (rc < 0) {
throw new EndOfStreamException(
"Unable to read additional data from client sessionid 0x"
+ Long.toHexString(sessionId)
+ ", likely client has closed socket");
}
if (incomingBuffer.remaining() == 0) {
boolean isPayload;
if (incomingBuffer == lenBuffer) { // start of next request
incomingBuffer.flip();
isPayload = readLength(k);
incomingBuffer.clear();
} else {
// continuation
isPayload = true;
}
if (isPayload) { // not the case for 4letterword
readPayload();
}
else {
// four letter words take care
// need not do anything else
return;
}
}
}
if (k.isWritable()) {
handleWrite(k);
if (!initialized && !getReadInterest() && !getWriteInterest()) {
throw new CloseRequestException("responded to info probe");
}
}
} catch (CancelledKeyException e) {
LOG.warn("CancelledKeyException causing close of session 0x"
+ Long.toHexString(sessionId));
if (LOG.isDebugEnabled()) {
LOG.debug("CancelledKeyException stack trace", e);
}
close();
} catch (CloseRequestException e) {
// expecting close to log session closure
close();
} catch (EndOfStreamException e) {
LOG.warn(e.getMessage());
// expecting close to log session closure
close();
} catch (IOException e) {
LOG.warn("Exception causing close of session 0x"
+ Long.toHexString(sessionId) + ": " + e.getMessage());
if (LOG.isDebugEnabled()) {
LOG.debug("IOException stack trace", e);
}
close();
}
}
private void readRequest() throws IOException {
zkServer.processPacket(this, incomingBuffer);
}
// Only called as callback from zkServer.processPacket()
protected void incrOutstandingRequests(RequestHeader h) {
if (h.getXid() >= 0) {
outstandingRequests.incrementAndGet();
// check throttling
int inProcess = zkServer.getInProcess();
if (inProcess > outstandingLimit) {
if (LOG.isDebugEnabled()) {
LOG.debug("Throttling recv " + inProcess);
}
disableRecv();
}
}
}
// returns whether we are interested in writing, which is determined
// by whether we have any pending buffers on the output queue or not
private boolean getWriteInterest() {
return !outgoingBuffers.isEmpty();
}
// returns whether we are interested in taking new requests, which is
// determined by whether we are currently throttled or not
private boolean getReadInterest() {
return !throttled.get();
}
private final AtomicBoolean throttled = new AtomicBoolean(false);
// Throttle acceptance of new requests. If this entailed a state change,
// register an interest op update request with the selector.
public void disableRecv() {
if (throttled.compareAndSet(false, true)) {
requestInterestOpsUpdate();
}
}
// Disable throttling and resume acceptance of new requests. If this
// entailed a state change, register an interest op update request with
// the selector.
public void enableRecv() {
if (throttled.compareAndSet(true, false)) {
requestInterestOpsUpdate();
}
}
private void readConnectRequest() throws IOException, InterruptedException {
if (!isZKServerRunning()) {
throw new IOException("ZooKeeperServer not running");
}
zkServer.processConnectRequest(this, incomingBuffer);
initialized = true;
}
/**
* This class wraps the sendBuffer method of NIOServerCnxn. It is
* responsible for chunking up the response to a client. Rather
* than cons'ing up a response fully in memory, which may be large
* for some commands, this class chunks up the result.
*/
private class SendBufferWriter extends Writer {
private StringBuffer sb = new StringBuffer();
/**
* Check if we are ready to send another chunk.
* @param force force sending, even if not a full chunk
*/
private void checkFlush(boolean force) {
if ((force && sb.length() > 0) || sb.length() > 2048) {
sendBufferSync(ByteBuffer.wrap(sb.toString().getBytes()));
// clear our internal buffer
sb.setLength(0);
}
}
@Override
public void close() throws IOException {
if (sb == null) return;
checkFlush(true);
sb = null; // clear out the ref to ensure no reuse
}
@Override
public void flush() throws IOException {
checkFlush(true);
}
@Override
public void write(char[] cbuf, int off, int len) throws IOException {
sb.append(cbuf, off, len);
checkFlush(false);
}
}
/** Return if four letter word found and responded to, otw false **/
private boolean checkFourLetterWord(final SelectionKey k, final int len)
throws IOException
{
// We take advantage of the limited size of the length to look
// for cmds. They are all 4-bytes which fits inside of an int
if (!FourLetterCommands.isKnown(len)) {
return false;
}
String cmd = FourLetterCommands.getCommandString(len);
packetReceived();
/** cancel the selection key to remove the socket handling
* from selector. This is to prevent netcat problem wherein
* netcat immediately closes the sending side after sending the
* commands and still keeps the receiving channel open.
* The idea is to remove the selectionkey from the selector
* so that the selector does not notice the closed read on the
* socket channel and keep the socket alive to write the data to
* and makes sure to close the socket after its done writing the data
*/
if (k != null) {
try {
k.cancel();
} catch(Exception e) {
LOG.error("Error cancelling command selection key ", e);
}
}
final PrintWriter pwriter = new PrintWriter(
new BufferedWriter(new SendBufferWriter()));
// ZOOKEEPER-2693: don't execute 4lw if it's not enabled.
if (!FourLetterCommands.isEnabled(cmd)) {
LOG.debug("Command {} is not executed because it is not in the whitelist.", cmd);
NopCommand nopCmd = new NopCommand(pwriter, this, cmd +
" is not executed because it is not in the whitelist.");
nopCmd.start();
return true;
}
LOG.info("Processing " + cmd + " command from "
+ sock.socket().getRemoteSocketAddress());
if (len == FourLetterCommands.setTraceMaskCmd) {
incomingBuffer = ByteBuffer.allocate(8);
int rc = sock.read(incomingBuffer);
if (rc < 0) {
throw new IOException("Read error");
}
incomingBuffer.flip();
long traceMask = incomingBuffer.getLong();
ZooTrace.setTextTraceLevel(traceMask);
SetTraceMaskCommand setMask = new SetTraceMaskCommand(pwriter, this, traceMask);
setMask.start();
return true;
} else {
CommandExecutor commandExecutor = new CommandExecutor();
return commandExecutor.execute(this, pwriter, len, zkServer, factory);
}
}
/** Reads the first 4 bytes of lenBuffer, which could be true length or
* four letter word.
*
* @param k selection key
* @return true if length read, otw false (wasn't really the length)
* @throws IOException if buffer size exceeds maxBuffer size
*/
private boolean readLength(SelectionKey k) throws IOException {
// Read the length, now get the buffer
int len = lenBuffer.getInt();
if (!initialized && checkFourLetterWord(sk, len)) {
return false;
}
if (len < 0 || len > BinaryInputArchive.maxBuffer) {
throw new IOException("Len error " + len);
}
if (!isZKServerRunning()) {
throw new IOException("ZooKeeperServer not running");
}
incomingBuffer = ByteBuffer.allocate(len);
return true;
}
/**
* @return true if the server is running, false otherwise.
*/
boolean isZKServerRunning() {
return zkServer != null && zkServer.isRunning();
}
public long getOutstandingRequests() {
return outstandingRequests.get();
}
/*
* (non-Javadoc)
*
* @see org.apache.zookeeper.server.ServerCnxnIface#getSessionTimeout()
*/
public int getSessionTimeout() {
return sessionTimeout;
}
/**
* Used by "dump" 4-letter command to list all connection in
* cnxnExpiryMap
*/
@Override
public String toString() {
return "ip: " + sock.socket().getRemoteSocketAddress() +
" sessionId: 0x" + Long.toHexString(sessionId);
}
/**
* Close the cnxn and remove it from the factory cnxns list.
*/
@Override
public void close() {
if (!factory.removeCnxn(this)) {
return;
}
if (zkServer != null) {
zkServer.removeCnxn(this);
}
if (sk != null) {
try {
// need to cancel this selection key from the selector
sk.cancel();
} catch (Exception e) {
if (LOG.isDebugEnabled()) {
LOG.debug("ignoring exception during selectionkey cancel", e);
}
}
}
closeSock();
}
/**
* Close resources associated with the sock of this cnxn.
*/
private void closeSock() {
if (sock.isOpen() == false) {
return;
}
LOG.info("Closed socket connection for client "
+ sock.socket().getRemoteSocketAddress()
+ (sessionId != 0 ?
" which had sessionid 0x" + Long.toHexString(sessionId) :
" (no session established for client)"));
closeSock(sock);
}
/**
* Close resources associated with a sock.
*/
public static void closeSock(SocketChannel sock) {
if (sock.isOpen() == false) {
return;
}
try {
/*
* The following sequence of code is stupid! You would think that
* only sock.close() is needed, but alas, it doesn't work that way.
* If you just do sock.close() there are cases where the socket
* doesn't actually close...
*/
sock.socket().shutdownOutput();
} catch (IOException e) {
// This is a relatively common exception that we can't avoid
if (LOG.isDebugEnabled()) {
LOG.debug("ignoring exception during output shutdown", e);
}
}
try {
sock.socket().shutdownInput();
} catch (IOException e) {
// This is a relatively common exception that we can't avoid
if (LOG.isDebugEnabled()) {
LOG.debug("ignoring exception during input shutdown", e);
}
}
try {
sock.socket().close();
} catch (IOException e) {
if (LOG.isDebugEnabled()) {
LOG.debug("ignoring exception during socket close", e);
}
}
try {
sock.close();
} catch (IOException e) {
if (LOG.isDebugEnabled()) {
LOG.debug("ignoring exception during socketchannel close", e);
}
}
}
private final static byte fourBytes[] = new byte[4];
/*
* (non-Javadoc)
*
* @see org.apache.zookeeper.server.ServerCnxnIface#sendResponse(org.apache.zookeeper.proto.ReplyHeader,
* org.apache.jute.Record, java.lang.String)
*/
@Override
public void sendResponse(ReplyHeader h, Record r, String tag) {
try {
ByteArrayOutputStream baos = new ByteArrayOutputStream();
// Make space for length
BinaryOutputArchive bos = BinaryOutputArchive.getArchive(baos);
try {
baos.write(fourBytes);
bos.writeRecord(h, "header");
if (r != null) {
bos.writeRecord(r, tag);
}
baos.close();
} catch (IOException e) {
LOG.error("Error serializing response");
}
byte b[] = baos.toByteArray();
ByteBuffer bb = ByteBuffer.wrap(b);
bb.putInt(b.length - 4).rewind();
sendBuffer(bb);
if (h.getXid() > 0) {
// check throttling
if (outstandingRequests.decrementAndGet() < 1 ||
zkServer.getInProcess() < outstandingLimit) {
enableRecv();
}
}
} catch(Exception e) {
LOG.warn("Unexpected exception. Destruction averted.", e);
}
}
/*
* (non-Javadoc)
*
* @see org.apache.zookeeper.server.ServerCnxnIface#process(org.apache.zookeeper.proto.WatcherEvent)
*/
@Override
public void process(WatchedEvent event) {
ReplyHeader h = new ReplyHeader(-1, -1L, 0);
if (LOG.isTraceEnabled()) {
ZooTrace.logTraceMessage(LOG, ZooTrace.EVENT_DELIVERY_TRACE_MASK,
"Deliver event " + event + " to 0x"
+ Long.toHexString(this.sessionId)
+ " through " + this);
}
// Convert WatchedEvent to a type that can be sent over the wire
WatcherEvent e = event.getWrapper();
sendResponse(h, e, "notification");
}
/*
* (non-Javadoc)
*
* @see org.apache.zookeeper.server.ServerCnxnIface#getSessionId()
*/
@Override
public long getSessionId() {
return sessionId;
}
@Override
public void setSessionId(long sessionId) {
this.sessionId = sessionId;
factory.addSession(sessionId, this);
}
@Override
public void setSessionTimeout(int sessionTimeout) {
this.sessionTimeout = sessionTimeout;
factory.touchCnxn(this);
}
@Override
public int getInterestOps() {
if (!isSelectable()) {
return 0;
}
int interestOps = 0;
if (getReadInterest()) {
interestOps |= SelectionKey.OP_READ;
}
if (getWriteInterest()) {
interestOps |= SelectionKey.OP_WRITE;
}
return interestOps;
}
@Override
public InetSocketAddress getRemoteSocketAddress() {
if (sock.isOpen() == false) {
return null;
}
return (InetSocketAddress) sock.socket().getRemoteSocketAddress();
}
public InetAddress getSocketAddress() {
if (sock.isOpen() == false) {
return null;
}
return sock.socket().getInetAddress();
}
@Override
protected ServerStats serverStats() {
if (zkServer == null) {
return null;
}
return zkServer.serverStats();
}
@Override
public boolean isSecure() {
return false;
}
@Override
public Certificate[] getClientCertificateChain() {
throw new UnsupportedOperationException(
"SSL is unsupported in NIOServerCnxn");
}
@Override
public void setClientCertificateChain(Certificate[] chain) {
throw new UnsupportedOperationException(
"SSL is unsupported in NIOServerCnxn");
}
}
| {'content_hash': '4539fded6eeb848aa16f35911d782beb', 'timestamp': '', 'source': 'github', 'line_count': 803, 'max_line_length': 108, 'avg_line_length': 34.0, 'alnum_prop': 0.5603985056039851, 'repo_name': 'JiangJiafu/zookeeper', 'id': '446438c5363bb87b3a32a5ef7c329f68b1841d18', 'size': '28108', 'binary': False, 'copies': '4', 'ref': 'refs/heads/master', 'path': 'src/java/main/org/apache/zookeeper/server/NIOServerCnxn.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Batchfile', 'bytes': '5969'}, {'name': 'C', 'bytes': '520877'}, {'name': 'C++', 'bytes': '673611'}, {'name': 'CMake', 'bytes': '6588'}, {'name': 'CSS', 'bytes': '4016'}, {'name': 'HTML', 'bytes': '40639'}, {'name': 'Java', 'bytes': '4272622'}, {'name': 'JavaScript', 'bytes': '239387'}, {'name': 'M4', 'bytes': '46802'}, {'name': 'Makefile', 'bytes': '10860'}, {'name': 'Mako', 'bytes': '13678'}, {'name': 'Perl', 'bytes': '33491'}, {'name': 'Perl 6', 'bytes': '115943'}, {'name': 'Python', 'bytes': '157028'}, {'name': 'Shell', 'bytes': '98150'}, {'name': 'XS', 'bytes': '66352'}, {'name': 'XSLT', 'bytes': '6024'}]} |
<?php
require_once 'PHPUnit/Util/Filter.php';
PHPUnit_Util_Filter::addFileToFilter(__FILE__);
require_once 'PHPUnit/Extensions/OutputTestCase.php';
/**
*
*
* @category Testing
* @package PHPUnit
* @author Sebastian Bergmann <[email protected]>
* @copyright 2002-2008 Sebastian Bergmann <[email protected]>
* @license http://www.opensource.org/licenses/bsd-license.php BSD License
* @version Release: 3.2.9
* @link http://www.phpunit.de/
* @since Class available since Release 2.0.0
*/
class OutputTestCase extends PHPUnit_Extensions_OutputTestCase
{
public function testExpectOutputStringFooActualFoo()
{
$this->expectOutputString('foo');
print 'foo';
}
public function testExpectOutputStringFooActualBar()
{
$this->expectOutputString('foo');
print 'bar';
}
public function testExpectOutputRegexFooActualFoo()
{
$this->expectOutputRegex('/foo/');
print 'foo';
}
public function testExpectOutputRegexFooActualBar()
{
$this->expectOutputRegex('/foo/');
print 'bar';
}
}
?>
| {'content_hash': 'eba7d138b6a9a31c2db4bb4e185da540', 'timestamp': '', 'source': 'github', 'line_count': 48, 'max_line_length': 78, 'avg_line_length': 23.833333333333332, 'alnum_prop': 0.6547202797202797, 'repo_name': 'nevali/shindig', 'id': '68abb7d54b8087e4810a2e318280fcefcbf1d1b6', 'size': '3222', 'binary': False, 'copies': '1', 'ref': 'refs/heads/0.8.1-x', 'path': 'php/external/PHPUnit/Tests/_files/OutputTestCase.php', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Java', 'bytes': '1313610'}, {'name': 'JavaScript', 'bytes': '525657'}, {'name': 'PHP', 'bytes': '856857'}, {'name': 'Shell', 'bytes': '6919'}]} |
""" Models for display visual shapes whose attributes can be associated
with data columns from data sources.
"""
from __future__ import absolute_import
from ..plot_object import PlotObject
from ..mixins import FillProps, LineProps, TextProps
from ..enums import Direction, Anchor
from ..properties import AngleSpec, Bool, DistanceSpec, Enum, Float, Include, Instance, NumberSpec, StringSpec
from .mappers import LinearColorMapper
class Glyph(PlotObject):
""" Base class for all glyphs/marks/geoms/whatever-you-call-'em in Bokeh.
"""
visible = Bool(help="""
Whether the glyph should render or not.
""")
class AnnularWedge(Glyph):
""" Render annular wedges.
Example
-------
.. bokeh-plot:: ../tests/glyphs/AnnularWedge.py
:source-position: none
*source:* `tests/glyphs/AnnularWedge.py <https://github.com/bokeh/bokeh/tree/master/tests/glyphs/AnnularWedge.py>`_
"""
x = NumberSpec("x", help="""
The x-coordinates of the center of the annular wedges.
""")
y = NumberSpec("y", help="""
The y-coordinates of the center of the annular wedges.
""")
inner_radius = DistanceSpec("inner_radius", help="""
The inner radii of the annular wedges.
""")
outer_radius = DistanceSpec("outer_radius", help="""
The outer radii of the annular wedges.
""")
start_angle = AngleSpec("start_angle", help="""
The angles to start the annular wedges, in radians, as measured from
the horizontal.
""")
end_angle = AngleSpec("end_angle", help="""
The angles to end the annular wedges, in radians, as measured from
the horizontal.
""")
direction = Enum(Direction, help="""
Which direction to stroke between the start and end angles.
""")
line_props = Include(LineProps, use_prefix=False, help="""
The %s values for the annular wedges.
""")
fill_props = Include(FillProps, use_prefix=False, help="""
The %s values for the annular wedges.
""")
class Annulus(Glyph):
""" Render annuli.
Example
-------
.. bokeh-plot:: ../tests/glyphs/Annulus.py
:source-position: none
*source:* `tests/glyphs/Annulus.py <https://github.com/bokeh/bokeh/tree/master/tests/glyphs/Annulus.py>`_
"""
x = NumberSpec("x", help="""
The x-coordinates of the center of the annuli.
""")
y = NumberSpec("y", help="""
The y-coordinates of the center of the annuli.
""")
inner_radius = DistanceSpec("inner_radius", help="""
The inner radii of the annuli.
""")
outer_radius = DistanceSpec("outer_radius", help="""
The outer radii of the annuli.
""")
line_props = Include(LineProps, use_prefix=False, help="""
The %s values for the annuli.
""")
fill_props = Include(FillProps, use_prefix=False, help="""
The %s values for the annuli.
""")
class Arc(Glyph):
""" Render arcs.
Example
-------
.. bokeh-plot:: ../tests/glyphs/Arc.py
:source-position: none
*source:* `tests/glyphs/Arc.py <https://github.com/bokeh/bokeh/tree/master/tests/glyphs/Arc.py>`_
"""
x = NumberSpec("x", help="""
The x-coordinates of the center of the arcs.
""")
y = NumberSpec("y", help="""
The y-coordinates of the center of the arcs.
""")
radius = DistanceSpec("radius", help="""
Radius of the arc.
""")
start_angle = AngleSpec("start_angle", help="""
The angles to start the arcs, in radians, as measured from the horizontal.
""")
end_angle = AngleSpec("end_angle", help="""
The angles to end the arcs, in radians, as measured from the horizontal.
""")
direction = Enum(Direction, help="""
Which direction to stroke between the start and end angles.
""")
line_props = Include(LineProps, use_prefix=False, help="""
The %s values for the arcs.
""")
class Bezier(Glyph):
u""" Render Bézier curves.
For more information consult the `Wikipedia article for Bézier curve`_.
.. _Wikipedia article for Bézier curve: http://en.wikipedia.org/wiki/Bézier_curve
Example
-------
.. bokeh-plot:: ../tests/glyphs/Bezier.py
:source-position: none
*source:* `tests/glyphs/Bezier.py <https://github.com/bokeh/bokeh/tree/master/tests/glyphs/Bezier.py>`_
"""
x0 = NumberSpec("x0", help="""
The x-coordinates of the starting points.
""")
y0 = NumberSpec("y0", help="""
The y-coordinates of the starting points.
""")
x1 = NumberSpec("x1", help="""
The x-coordinates of the ending points.
""")
y1 = NumberSpec("y1", help="""
The y-coordinates of the ending points.
""")
cx0 = NumberSpec("cx0", help="""
The x-coordinates of first control points.
""")
cy0 = NumberSpec("cy0", help="""
The y-coordinates of first control points.
""")
cx1 = NumberSpec("cx1", help="""
The x-coordinates of second control points.
""")
cy1 = NumberSpec("cy1", help="""
The y-coordinates of second control points.
""")
line_props = Include(LineProps, use_prefix=False, help=u"""
The %s values for the Bézier curves.
""")
class Gear(Glyph):
""" Render gears.
The details and nomenclature concerning gear construction can
be quite involved. For more information, consult the `Wikipedia
article for Gear`_.
.. _Wikipedia article for Gear: http://en.wikipedia.org/wiki/Gear
Example
-------
.. bokeh-plot:: ../tests/glyphs/Gear.py
:source-position: none
*source:* `tests/glyphs/Gear.py <https://github.com/bokeh/bokeh/tree/master/tests/glyphs/Gear.py>`_
"""
x = NumberSpec("x", help="""
The x-coordinates of the center of the gears.
""")
y = NumberSpec("y", help="""
The y-coordinates of the center of the gears.
""")
angle = AngleSpec(default=0, help="""
The angle the gears are rotated from horizontal. [rad]
""")
module = NumberSpec("module", help="""
A scaling factor, given by::
m = p / pi
where *p* is the circular pitch, defined as the distance from one
face of a tooth to the corresponding face of an adjacent tooth on
the same gear, measured along the pitch circle. [float]
""")
teeth = NumberSpec("teeth", help="""
How many teeth the gears have. [int]
""")
pressure_angle = NumberSpec(default=20, help= """
The complement of the angle between the direction that the teeth
exert force on each other, and the line joining the centers of the
two gears. [deg]
""")
# TODO: (bev) evidently missing a test for default value
shaft_size = NumberSpec(default=0.3, help="""
The central gear shaft size as a percentage of the overall gear
size. [float]
""")
# TODO: (bev) evidently missing a test for default value
internal = NumberSpec(default=False, help="""
Whether the gear teeth are internal. [bool]
""")
line_props = Include(LineProps, use_prefix=False, help="""
The %s values for the gears.
""")
fill_props = Include(FillProps, use_prefix=False, help="""
The %s values for the gears.
""")
class Image(Glyph):
""" Render images given as scalar data together with a color
mapper.
"""
def __init__(self, **kwargs):
if 'palette' in kwargs and 'color_mapper' in kwargs:
raise ValueError("only one of 'palette' and 'color_mapper' may be specified")
elif 'color_mapper' not in kwargs:
# Use a palette (given or default)
palette = kwargs.pop('palette', 'Greys9')
mapper = LinearColorMapper(palette)
reserve_val = kwargs.pop('reserve_val', None)
if reserve_val is not None:
mapper.reserve_val = reserve_val
reserve_color = kwargs.pop('reserve_color', None)
if reserve_color is not None:
mapper.reserve_color = reserve_color
kwargs['color_mapper'] = mapper
super(Image, self).__init__(**kwargs)
image = NumberSpec("image", help="""
The arrays of scalar data for the images to be colormapped.
""")
x = NumberSpec("x", help="""
The x-coordinates to locate the image anchors.
""")
y = NumberSpec("y", help="""
The y-coordinates to locate the image anchors.
""")
dw = DistanceSpec("dw", help="""
The widths of the plot regions that the images will occupy.
.. note::
This is not the number of pixels that an image is wide.
That number is fixed by the image itself.
""")
dh = DistanceSpec("dh", help="""
The height of the plot region that the image will occupy.
.. note::
This is not the number of pixels that an image is tall.
That number is fixed by the image itself.
""")
dilate = Bool(False, help="""
Whether to always round fractional pixel locations in such a way
as to make the images bigger.
This setting may be useful if pixel rounding errors are causing
images to have a gap between them, when they should appear flush.
""")
color_mapper = Instance(LinearColorMapper, help="""
A ``ColorMapper`` to use to map the scalar data from ``image``
into RGBA values for display.
.. note::
The color mapping step happens on the client.
""")
# TODO: (bev) support anchor property for Image
# ref: https://github.com/bokeh/bokeh/issues/1763
class ImageRGBA(Glyph):
""" Render images given as RGBA data.
"""
image = NumberSpec("image", help="""
The arrays of RGBA data for the images.
""")
x = NumberSpec("x", help="""
The x-coordinates to locate the image anchors.
""")
y = NumberSpec("y", help="""
The y-coordinates to locate the image anchors.
""")
rows = NumberSpec("rows", help="""
The numbers of rows in the images
""")
cols = NumberSpec("cols", help="""
The numbers of columns in the images
""")
dw = DistanceSpec("dw", help="""
The widths of the plot regions that the images will occupy.
.. note::
This is not the number of pixels that an image is wide.
That number is fixed by the image itself.
""")
dh = DistanceSpec("dh", help="""
The height of the plot region that the image will occupy.
.. note::
This is not the number of pixels that an image is tall.
That number is fixed by the image itself.
""")
dilate = Bool(False, help="""
Whether to always round fractional pixel locations in such a way
as to make the images bigger.
This setting may be useful if pixel rounding errors are causing
images to have a gap between them, when they should appear flush.
""")
# TODO: (bev) support anchor property for ImageRGBA
# ref: https://github.com/bokeh/bokeh/issues/1763
class ImageURL(Glyph):
""" Render images loaded from given URLs.
Example
-------
.. bokeh-plot:: ../tests/glyphs/ImageURL.py
:source-position: none
*source:* `tests/glyphs/ImageURL.py <https://github.com/bokeh/bokeh/tree/master/tests/glyphs/ImageURL.py>`_
"""
url = NumberSpec("url", help="""
The URLs to retrieve images from.
.. note::
The actual retrieving and loading of the images happens on
the client.
""")
x = NumberSpec("x", help="""
The x-coordinates to locate the image anchors.
""")
y = NumberSpec("y", help="""
The y-coordinates to locate the image anchors.
""")
# TODO: (bev) rename to "dw" for consistency
w = DistanceSpec("w", help="""
The widths of the plot regions that the images will occupy.
.. note::
This is not the number of pixels that an image is wide.
That number is fixed by the image itself.
.. note::
This may be renamed to "dw" in the future.
""")
# TODO: (bev) rename to "dh" for consistency
h = DistanceSpec("h", help="""
The height of the plot region that the image will occupy.
.. note::
This is not the number of pixels that an image is tall.
That number is fixed by the image itself.
.. note::
This may be renamed to "dh" in the future.
""")
angle = AngleSpec(default=0, help="""
The angles to rotate the images, in radians as measured from the
horizontal.
""")
global_alpha = Float(1.0, help="""
The opacity that each image is rendered with.
""")
dilate = Bool(False, help="""
Whether to always round fractional pixel locations in such a way
as to make the images bigger.
This setting may be useful if pixel rounding errors are causing
images to have a gap between them, when they should appear flush.
""")
anchor = Enum(Anchor, help="""
What position of the image should be anchored at the `x`, `y`
coordinates.
""")
class Line(Glyph):
""" Render a single line.
.. note::
The ``Line`` glyph is different from most other glyphs in that
the vector of values only produces one glyph on the Plot.
Example
-------
.. bokeh-plot:: ../tests/glyphs/Line.py
:source-position: none
*source:* `tests/glyphs/Line.py <https://github.com/bokeh/bokeh/tree/master/tests/glyphs/Line.py>`_
"""
x = NumberSpec("x", help="""
The x-coordinates for the points of the line.
""")
y = NumberSpec("y", help="""
The y-coordinates for the points of the line.
""")
line_props = Include(LineProps, use_prefix=False, help="""
The %s values for the line.
""")
class MultiLine(Glyph):
""" Render several lines.
.. note::
The data for the ``MultiLine`` glyph is different in that the
vector of values is not a vector of scalars. Rather, it is a
"list of lists".
Example
-------
.. bokeh-plot:: ../tests/glyphs/MultiLine.py
:source-position: none
*source:* `tests/glyphs/MultiLine.py <https://github.com/bokeh/bokeh/tree/master/tests/glyphs/MultiLine.py>`_
"""
xs = NumberSpec("xs", help="""
The x-coordinates for all the lines, given as a "list of lists".
""")
ys = NumberSpec("ys", help="""
The x-coordinates for all the lines, given as a "list of lists".
""")
line_props = Include(LineProps, use_prefix=False, help="""
The %s values for the lines.
""")
class Oval(Glyph):
u""" Render ovals.
.. note::
This glyph renders ovals using Bézier curves, which are similar,
but not identical to ellipses.
Example
-------
.. bokeh-plot:: ../tests/glyphs/Oval.py
:source-position: none
*source:* `tests/glyphs/Oval.py <https://github.com/bokeh/bokeh/tree/master/tests/glyphs/Oval.py>`_
"""
x = NumberSpec("x", help="""
The x-coordinates of the centers of the ovals.
""")
y = NumberSpec("y", help="""
The y-coordinates of the centers of the ovals.
""")
width = DistanceSpec("width", help="""
The overall widths of each oval.
""")
height = DistanceSpec("height", help="""
The overall height of each oval.
""")
angle = AngleSpec("angle", help="""
The angle the ovals are rotated from horizontal. [rad]
""")
line_props = Include(LineProps, use_prefix=False, help="""
The %s values for the ovals.
""")
fill_props = Include(FillProps, use_prefix=False, help="""
The %s values for the ovals.
""")
class Patch(Glyph):
""" Render a single patch.
.. note::
The ``Patch`` glyph is different from most other glyphs in that
the vector of values only produces one glyph on the Plot.
Example
-------
.. bokeh-plot:: ../tests/glyphs/Patch.py
:source-position: none
*source:* `tests/glyphs/Patch.py <https://github.com/bokeh/bokeh/tree/master/tests/glyphs/Patch.py>`_
"""
x = NumberSpec("x", help="""
The x-coordinates for the points of the patch.
.. note::
A patch may comprise multiple polygons. In this case the
x-coordinates for each polygon should be separated by NaN
values in the sequence.
""")
y = NumberSpec("y", help="""
The y-coordinates for the points of the patch.
.. note::
A patch may comprise multiple polygons. In this case the
y-coordinates for each polygon should be separated by NaN
values in the sequence.
""")
line_props = Include(LineProps, use_prefix=False, help="""
The %s values for the patch.
""")
fill_props = Include(FillProps, use_prefix=False, help="""
The %s values for the patch.
""")
class Patches(Glyph):
""" Render several patches.
.. note::
The data for the ``Patches`` glyph is different in that the
vector of values is not a vector of scalars. Rather, it is a
"list of lists".
Example
-------
.. bokeh-plot:: ../tests/glyphs/Patches.py
:source-position: none
*source:* `tests/glyphs/Patches.py <https://github.com/bokeh/bokeh/tree/master/tests/glyphs/Patches.py>`_
"""
xs = NumberSpec("xs", help="""
The x-coordinates for all the patches, given as a "list of lists".
.. note::
Individual patches may comprise multiple polygons. In this case
the x-coordinates for each polygon should be separated by NaN
values in the sublists.
""")
ys = NumberSpec("ys", help="""
The y-coordinates for all the patches, given as a "list of lists".
.. note::
Individual patches may comprise multiple polygons. In this case
the y-coordinates for each polygon should be separated by NaN
values in the sublists.
""")
line_props = Include(LineProps, use_prefix=False, help="""
The %s values for the patches.
""")
fill_props = Include(FillProps, use_prefix=False, help="""
The %s values for the patches.
""")
class Quad(Glyph):
""" Render axis-aligned quads.
Example
-------
.. bokeh-plot:: ../tests/glyphs/Quad.py
:source-position: none
*source:* `tests/glyphs/Quad.py <https://github.com/bokeh/bokeh/tree/master/tests/glyphs/Quad.py>`_
"""
left = NumberSpec("left", help="""
The x-coordinates of the left edges.
""")
right = NumberSpec("right", help="""
The x-coordinates of the right edges.
""")
bottom = NumberSpec("bottom", help="""
The y-coordinates of the bottom edges.
""")
top = NumberSpec("top", help="""
The y-coordinates of the top edges.
""")
line_props = Include(LineProps, use_prefix=False, help="""
The %s values for the quads.
""")
fill_props = Include(FillProps, use_prefix=False, help="""
The %s values for the quads.
""")
class Quadratic(Glyph):
""" Render parabolas.
Example
-------
.. bokeh-plot:: ../tests/glyphs/Quadratic.py
:source-position: none
*source:* `tests/glyphs/Quadratic.py <https://github.com/bokeh/bokeh/tree/master/tests/glyphs/Quadratic.py>`_
"""
x0 = NumberSpec("x0", help="""
The x-coordinates of the starting points.
""")
y0 = NumberSpec("y0", help="""
The y-coordinates of the starting points.
""")
x1 = NumberSpec("x1", help="""
The x-coordinates of the ending points.
""")
y1 = NumberSpec("y1", help="""
The y-coordinates of the ending points.
""")
cx = NumberSpec("cx", help="""
The x-coordinates of the control points.
""")
cy = NumberSpec("cy", help="""
The y-coordinates of the control points.
""")
line_props = Include(LineProps, use_prefix=False, help="""
The %s values for the parabolas.
""")
class Ray(Glyph):
""" Render rays.
Example
-------
.. bokeh-plot:: ../tests/glyphs/Ray.py
:source-position: none
*source:* `tests/glyphs/Ray.py <https://github.com/bokeh/bokeh/tree/master/tests/glyphs/Ray.py>`_
"""
x = NumberSpec("x", help="""
The x-coordinates to start the rays.
""")
y = NumberSpec("y", help="""
The y-coordinates to start the rays.
""")
angle = AngleSpec("angle", help="""
The angles in radians to extend the rays, as measured from the
horizontal.
""")
length = DistanceSpec("length", help="""
The length to extend the ray. Note that this ``length`` defaults
to screen units.
""")
line_props = Include(LineProps, use_prefix=False, help="""
The %s values for the rays.
""")
class Rect(Glyph):
""" Render rectangles.
Example
-------
.. bokeh-plot:: ../tests/glyphs/Rect.py
:source-position: none
*source:* `tests/glyphs/Rect.py <https://github.com/bokeh/bokeh/tree/master/tests/glyphs/Rect.py>`_
"""
x = NumberSpec("x", help="""
The x-coordinates of the centers of the rectangles.
""")
y = NumberSpec("y", help="""
The y-coordinates of the centers of the rectangles.
""")
width = DistanceSpec("width", help="""
The overall widths of the rectangles.
""")
height = DistanceSpec("height", help="""
The overall heights of the rectangles.
""")
angle = AngleSpec("angle", help="""
The angles to rotate the rectangles, in radians, as measured from
the horizontal.
""")
dilate = Bool(False, help="""
Whether to always round fractional pixel locations in such a way
as to make the rectangles bigger.
This setting may be useful if pixel rounding errors are causing
rectangles to have a gap between them, when they should appear
flush.
""")
line_props = Include(LineProps, use_prefix=False, help="""
The %s values for the rectangles.
""")
fill_props = Include(FillProps, use_prefix=False, help="""
The %s values for the rectangles.
""")
class Segment(Glyph):
""" Render segments.
Example
-------
.. bokeh-plot:: ../tests/glyphs/Segment.py
:source-position: none
*source:* `tests/glyphs/Segment.py <https://github.com/bokeh/bokeh/tree/master/tests/glyphs/Segment.py>`_
"""
x0 = NumberSpec("x0", help="""
The x-coordinates of the starting points.
""")
y0 = NumberSpec("y0", help="""
The y-coordinates of the starting points.
""")
x1 = NumberSpec("x1", help="""
The x-coordinates of the ending points.
""")
y1 = NumberSpec("y1", help="""
The y-coordinates of the ending points.
""")
line_props = Include(LineProps, use_prefix=False, help="""
The %s values for the segments.
""")
class Text(Glyph):
""" Render text.
Example
-------
.. bokeh-plot:: ../tests/glyphs/Text.py
:source-position: none
*source:* `tests/glyphs/Text.py <https://github.com/bokeh/bokeh/tree/master/tests/glyphs/Text.py>`_
"""
x = NumberSpec("x", help="""
The x-coordinates to locate the text anchors.
""")
y = NumberSpec("y", help="""
The y-coordinates to locate the text anchors.
""")
text = StringSpec("text", help="""
The text values to render.
""")
angle = AngleSpec(default=0, help="""
The angles to rotate the text, in radians,, as measured from the horizontal.
""")
x_offset = NumberSpec(default=0, help="""
Offset values to apply to the x-coordinates.
This is useful, for instance, if it is desired to "float" text a fixed
distance in screen units from a given data position.
""")
y_offset = NumberSpec(default=0, help="""
Offset values to apply to the y-coordinates.
This is useful, for instance, if it is desired to "float" text a fixed
distance in screen units from a given data position.
""")
text_props = Include(TextProps, use_prefix=False, help="""
The %s values for the text.
""")
class Wedge(Glyph):
""" Render wedges.
Example
-------
.. bokeh-plot:: ../tests/glyphs/Wedge.py
:source-position: none
*source:* `tests/glyphs/Wedge.py <https://github.com/bokeh/bokeh/tree/master/tests/glyphs/Wedge.py>`_
"""
x = NumberSpec("x", help="""
The x-coordinates of the points of the wedges.
""")
y = NumberSpec("y", help="""
The y-coordinates of the points of the wedges.
""")
radius = DistanceSpec("radius", help="""
Radii of the wedges.
""")
start_angle = AngleSpec("start_angle", help="""
The angles to start the wedges, in radians, as measured from the horizontal.
""")
end_angle = AngleSpec("end_angle", help="""
The angles to end the wedges, in radians as measured from the horizontal.
""")
direction = Enum(Direction, help="""
Which direction to stroke between the start and end angles.
""")
line_props = Include(LineProps, use_prefix=False, help="""
The %s values for the wedges.
""")
fill_props = Include(FillProps, use_prefix=False, help="""
The %s values for the wedges.
""")
# XXX: allow `from bokeh.models.glyphs import *`
from .markers import (Marker, Asterisk, Circle, CircleCross, CircleX, Cross,
Diamond, DiamondCross, InvertedTriangle, Square,
SquareCross, SquareX, Triangle, X)
# Fool pyflakes
(Marker, Asterisk, Circle, CircleCross, CircleX, Cross, Diamond, DiamondCross,
InvertedTriangle, Square, SquareCross, SquareX, Triangle, X)
| {'content_hash': '2fb1e55ec163f4d95798df477413a121', 'timestamp': '', 'source': 'github', 'line_count': 973, 'max_line_length': 119, 'avg_line_length': 26.082219938335047, 'alnum_prop': 0.6164788399401055, 'repo_name': 'daodaoliang/bokeh', 'id': 'cd15e0575fe6415967abbe568934fda1d0eb3ac8', 'size': '25408', 'binary': False, 'copies': '5', 'ref': 'refs/heads/master', 'path': 'bokeh/models/glyphs.py', 'mode': '33188', 'license': 'bsd-3-clause', 'language': [{'name': 'Batchfile', 'bytes': '5455'}, {'name': 'CSS', 'bytes': '413395'}, {'name': 'CoffeeScript', 'bytes': '1995470'}, {'name': 'HTML', 'bytes': '1545838'}, {'name': 'JavaScript', 'bytes': '4747'}, {'name': 'Makefile', 'bytes': '5785'}, {'name': 'Python', 'bytes': '1381168'}, {'name': 'Shell', 'bytes': '13857'}]} |
stage: Verify
group: Testing
info: To determine the technical writer assigned to the Stage/Group associated with this page, see https://about.gitlab.com/handbook/engineering/ux/technical-writing/#designated-technical-writers
type: reference, howto
---
# Load Performance Testing **(PREMIUM)**
> [Introduced](https://gitlab.com/gitlab-org/gitlab/-/issues/10683) in [GitLab Premium](https://about.gitlab.com/pricing/) 13.2.
With Load Performance Testing, you can test the impact of any pending code changes
to your application's backend in [GitLab CI/CD](../../../ci/README.md).
GitLab uses [k6](https://k6.io/), a free and open source
tool, for measuring the system performance of applications under
load.
Unlike [Browser Performance Testing](browser_performance_testing.md), which is
used to measure how web sites perform in client browsers, Load Performance Testing
can be used to perform various types of [load tests](https://k6.io/docs/#use-cases)
against application endpoints such as APIs, Web Controllers, and so on.
This can be used to test how the backend or the server performs at scale.
For example, you can use Load Performance Testing to perform many concurrent
GET calls to a popular API endpoint in your application to see how it performs.
## How Load Performance Testing works
First, define a job in your `.gitlab-ci.yml` file that generates the
[Load Performance report artifact](../../../ci/pipelines/job_artifacts.md#artifactsreportsload_performance-premium).
GitLab checks this report, compares key load performance metrics
between the source and target branches, and then shows the information in a merge request widget:

Next, you need to configure the test environment and write the k6 test.
The key performance metrics that the merge request widget shows after the test completes are:
- Checks: The percentage pass rate of the [checks](https://k6.io/docs/using-k6/checks) configured in the k6 test.
- TTFB P90: The 90th percentile of how long it took to start receiving responses, aka the [Time to First Byte](https://en.wikipedia.org/wiki/Time_to_first_byte) (TTFB).
- TTFB P95: The 95th percentile for TTFB.
- RPS: The average requests per second (RPS) rate the test was able to achieve.
NOTE: **Note:**
If the Load Performance report has no data to compare, such as when you add the
Load Performance job in your `.gitlab-ci.yml` for the very first time,
the Load Performance report widget won't show. It must have run at least
once on the target branch (`master`, for example), before it will display in a
merge request targeting that branch.
## Configure the Load Performance Testing job
Configuring your Load Performance Testing job can be broken down into several distinct parts:
- Determine the test parameters such as throughput, and so on.
- Set up the target test environment for load performance testing.
- Design and write the k6 test.
### Determine the test parameters
The first thing you need to do is determine the [type of load test](https://k6.io/docs/test-types/introduction)
you want to run, and how it will run (for example, the number of users, throughput, and so on).
Refer to the [k6 docs](https://k6.io/docs/), especially the [k6 testing guides](https://k6.io/docs/testing-guides),
for guidance on the above and more.
### Test Environment setup
A large part of the effort around load performance testing is to prepare the target test environment
for high loads. You should ensure it's able to handle the
[throughput](https://k6.io/blog/monthly-visits-concurrent-users) it will be tested with.
It's also typically required to have representative test data in the target environment
for the load performance test to use.
We strongly recommend [not running these tests against a production environment](https://k6.io/our-beliefs#load-test-in-a-pre-production-environment).
### Write the load performance test
After the environment is prepared, you can write the k6 test itself. k6 is a flexible
tool and can be used to run [many kinds of performance tests](https://k6.io/docs/test-types/introduction).
Refer to the [k6 documentation](https://k6.io/docs/) for detailed information on how to write tests.
### Configure the test in GitLab CI/CD
When your k6 test is ready, the next step is to configure the load performance
testing job in GitLab CI/CD. The easiest way to do this is to use the
[`Verify/Load-Performance-Testing.gitlab-ci.yml`](https://gitlab.com/gitlab-org/gitlab/blob/master/lib/gitlab/ci/templates/Verify/Load-Performance-Testing.gitlab-ci.yml)
template that is included with GitLab.
NOTE: **Note:**
For large scale k6 tests you need to ensure the GitLab Runner instance performing the actual
test is able to handle running the test. Refer to [k6's guidance](https://k6.io/docs/testing-guides/running-large-tests#hardware-considerations)
for spec details. The [default shared GitLab.com runners](../../gitlab_com/#linux-shared-runners)
likely have insufficient specs to handle most large k6 tests.
This template runs the
[k6 Docker container](https://hub.docker.com/r/loadimpact/k6/) in the job and provides several ways to customize the
job.
An example configuration workflow:
1. Set up a GitLab Runner that can run Docker containers, such as a Runner using the
[Docker-in-Docker workflow](../../../ci/docker/using_docker_build.md#use-docker-in-docker-workflow-with-docker-executor).
1. Configure the default Load Performance Testing CI job in your `.gitlab-ci.yml` file.
You need to include the template and configure it with variables:
```yaml
include:
template: Verify/Load-Performance-Testing.gitlab-ci.yml
load_performance:
variables:
K6_TEST_FILE: <PATH TO K6 TEST FILE IN PROJECT>
```
The above example creates a `load_performance` job in your CI/CD pipeline that runs
the k6 test.
NOTE: **Note:**
For Kubernetes setups a different template should be used: [`Jobs/Load-Performance-Testing.gitlab-ci.yml`](https://gitlab.com/gitlab-org/gitlab/blob/master/lib/gitlab/ci/templates/Jobs/Load-Performance-Testing.gitlab-ci.yml).
k6 has [various options](https://k6.io/docs/using-k6/options) to configure how it will run tests, such as what throughput (RPS) to run with,
how long the test should run, and so on. Almost all options can be configured in the test itself, but as
you can also pass command line options via the `K6_OPTIONS` variable.
For example, you can override the duration of the test with a CLI option:
```yaml
include:
template: Verify/Load-Performance-Testing.gitlab-ci.yml
load_performance:
variables:
K6_TEST_FILE: <PATH TO K6 TEST FILE IN PROJECT>
K6_OPTIONS: '--duration 30s'
```
GitLab only displays the key performance metrics in the MR widget if k6's results are saved
via [summary export](https://k6.io/docs/results-visualization/json#summary-export)
as a [Load Performance report artifact](../../../ci/pipelines/job_artifacts.md#artifactsreportsload_performance-premium).
The latest Load Performance artifact available is always used, using the
summary values from the test.
If [GitLab Pages](../pages/index.md) is enabled, you can view the report directly in your browser.
### Load Performance testing in Review Apps
The CI/CD YAML configuration example above works for testing against static environments,
but it can be extended to work with [review apps](../../../ci/review_apps) or
[dynamic environments](../../../ci/environments) with a few extra steps.
The best approach is to capture the dynamic URL into a custom environment variable that
is then [inherited](../../../ci/variables/README.md#inherit-environment-variables)
by the `load_performance` job. The k6 test script to be run should then be configured to
use that environment URL, such as: ``http.get(`${__ENV.ENVIRONMENT_URL`})``.
For example:
1. In the `review` job:
1. Capture the dynamic URL and save it into a `.env` file, e.g. `echo "ENVIRONMENT_URL=$CI_ENVIRONMENT_URL" >> review.env`.
1. Set the `.env` file to be an [`artifacts:reports:dotenv` report](../../../ci/variables/README.md#inherit-environment-variables).
1. Set the `load_performance` job to depend on the review job, so it inherits the environment variable.
1. Configure the k6 test script to use the environment variable in it's steps.
Your `.gitlab-ci.yml` file might be similar to:
```yaml
stages:
- deploy
- performance
include:
template: Verify/Load-Performance-Testing.gitlab-ci.yml
review:
stage: deploy
environment:
name: review/$CI_COMMIT_REF_NAME
url: http://$CI_ENVIRONMENT_SLUG.example.com
script:
- run_deploy_script
- echo "ENVIRONMENT_URL=$CI_ENVIRONMENT_URL" >> review.env
artifacts:
reports:
dotenv:
review.env
rules:
- if: '$CI_COMMIT_BRANCH' # Modify to match your pipeline rules, or use `only/except` if needed.
load_performance:
dependencies:
- review
rules:
- if: '$CI_COMMIT_BRANCH' # Modify to match your pipeline rules, or use `only/except` if needed.
```
| {'content_hash': '675d5958436694ac01e56f97ad21f2c6', 'timestamp': '', 'source': 'github', 'line_count': 197, 'max_line_length': 225, 'avg_line_length': 45.9492385786802, 'alnum_prop': 0.7568493150684932, 'repo_name': 'mmkassem/gitlabhq', 'id': '97f4f202ab376e7a67e7fbf50bda0412315358d7', 'size': '9056', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'doc/user/project/merge_requests/load_performance_testing.md', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '113683'}, {'name': 'CoffeeScript', 'bytes': '139197'}, {'name': 'Cucumber', 'bytes': '119759'}, {'name': 'HTML', 'bytes': '447030'}, {'name': 'JavaScript', 'bytes': '29805'}, {'name': 'Ruby', 'bytes': '2417833'}, {'name': 'Shell', 'bytes': '14336'}]} |
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import {DashboardComponent} from './dashboard.component';
const routes: Routes = [
{
path: '',
component: DashboardComponent
}
];
@NgModule({
imports: [RouterModule.forChild(routes)],
exports: [RouterModule]
})
export class DashboardRoutingModule { }
| {'content_hash': 'eae6b9a2be06708b54bbe807aa5a233f', 'timestamp': '', 'source': 'github', 'line_count': 16, 'max_line_length': 57, 'avg_line_length': 22.9375, 'alnum_prop': 0.7002724795640327, 'repo_name': 'aaronfurtado93/pwa-core', 'id': 'ee7ab816e54c01c739a14f328bf3f3639a9f78a5', 'size': '367', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/app/pages/dashboard/dashboard-routing.module.ts', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '2373'}, {'name': 'HTML', 'bytes': '7291'}, {'name': 'JavaScript', 'bytes': '1645'}, {'name': 'TypeScript', 'bytes': '56424'}]} |
var soundMap = [{
name: 'river water',
url: "http://hifi-production.s3.amazonaws.com/DomainContent/Toybox/sounds/Water_Lap_River_Edge_Gentle.L.wav",
audioOptions: {
position: {
x: 580,
y: 493,
z: 528
},
volume: 0.4,
loop: true
}
}, {
name: 'windmill',
url: "http://hifi-production.s3.amazonaws.com/DomainContent/Toybox/sounds/WINDMILL_Mono.wav",
audioOptions: {
position: {
x: 530,
y: 516,
z: 518
},
volume: 0.08,
loop: true
}
}, {
name: 'insects',
url: "http://hifi-production.s3.amazonaws.com/DomainContent/Toybox/sounds/insects3.wav",
audioOptions: {
position: {
x: 560,
y: 495,
z: 474
},
volume: 0.25,
loop: true
}
}, {
name: 'fireplace',
url: "http://hifi-production.s3.amazonaws.com/DomainContent/Toybox/sounds/0619_Fireplace__Tree_B.L.wav",
audioOptions: {
position: {
x: 551.61,
y: 494.88,
z: 502.00
},
volume: 0.25,
loop: true
}
}, {
name: 'cat purring',
url: "http://hifi-production.s3.amazonaws.com/DomainContent/Toybox/sounds/Cat_Purring_Deep_Low_Snor.wav",
audioOptions: {
position: {
x: 551.48,
y: 495.60,
z: 502.08
},
volume: 0.03,
loop: true
}
}, {
name: 'dogs barking',
url: "http://hifi-production.s3.amazonaws.com/DomainContent/Toybox/sounds/dogs_barking_1.L.wav",
audioOptions: {
position: {
x: 523,
y: 494.88,
z: 469
},
volume: 0.05,
loop: false
},
playAtInterval: 60 * 1000
}, {
name: 'arcade game',
url: "http://hifi-production.s3.amazonaws.com/DomainContent/Toybox/sounds/ARCADE_GAMES_VID.L.L.wav",
audioOptions: {
position: {
x: 543.77,
y: 495.07,
z: 502.25
},
volume: 0.01,
loop: false,
},
playAtInterval: 90 * 1000
}];
function loadSounds() {
soundMap.forEach(function(soundData) {
soundData.sound = SoundCache.getSound(soundData.url);
});
}
function playSound(soundData) {
if (soundData.injector) {
// try/catch in case the injector QObject has been deleted already
try {
soundData.injector.stop();
} catch (e) {}
}
soundData.injector = Audio.playSound(soundData.sound, soundData.audioOptions);
}
function checkDownloaded(soundData) {
if (soundData.sound.downloaded) {
Script.clearInterval(soundData.downloadTimer);
if (soundData.hasOwnProperty('playAtInterval')) {
soundData.playingInterval = Script.setInterval(function() {
playSound(soundData)
}, soundData.playAtInterval);
} else {
playSound(soundData);
}
}
}
function startCheckDownloadedTimers() {
soundMap.forEach(function(soundData) {
soundData.downloadTimer = Script.setInterval(function() {
checkDownloaded(soundData);
}, 1000);
});
}
Script.scriptEnding.connect(function() {
soundMap.forEach(function(soundData) {
if (soundData.hasOwnProperty("injector")) {
soundData.injector.stop();
}
if (soundData.hasOwnProperty("downloadTimer")) {
Script.clearInterval(soundData.downloadTimer);
}
if (soundData.hasOwnProperty("playingInterval")) {
Script.clearInterval(soundData.playingInterval);
}
});
});
loadSounds();
startCheckDownloadedTimers(); | {'content_hash': '7591a315854d23c17be8ef168571926c', 'timestamp': '', 'source': 'github', 'line_count': 149, 'max_line_length': 113, 'avg_line_length': 24.973154362416107, 'alnum_prop': 0.5530771298038162, 'repo_name': 'misslivirose/hifi-content', 'id': 'e73630e38068ba37528ceb120332fc10312fa904', 'size': '4031', 'binary': False, 'copies': '2', 'ref': 'refs/heads/master', 'path': 'DomainContent/Toybox/AC_scripts/toybox_sounds.js', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'CSS', 'bytes': '98041'}, {'name': 'GLSL', 'bytes': '3603'}, {'name': 'HTML', 'bytes': '316682'}, {'name': 'JavaScript', 'bytes': '3778239'}, {'name': 'Makefile', 'bytes': '317'}]} |
#include "types.h"
#include "qpid/Msg.h"
#include "qpid/broker/Message.h"
#include "qpid/broker/Queue.h"
#include "qpid/Exception.h"
#include <algorithm>
#include <iostream>
#include <iterator>
#include <assert.h>
namespace qpid {
namespace ha {
using namespace std;
const string QPID_REPLICATE("qpid.replicate");
const string QPID_HA_UUID("qpid.ha-uuid");
const char* QPID_HA_PREFIX = "qpid.ha-";
const char* QUEUE_REPLICATOR_PREFIX = "qpid.ha-q:";
bool startsWith(const string& name, const string& prefix) {
return name.compare(0, prefix.size(), prefix) == 0;
}
string EnumBase::str() const {
assert(value < count);
return names[value];
}
void EnumBase::parse(const string& s) {
if (!parseNoThrow(s))
throw Exception(QPID_MSG("Invalid " << name << " value: " << s));
}
bool EnumBase::parseNoThrow(const string& s) {
const char** i = find(names, names+count, s);
value = i - names;
return value < count;
}
template <> const char* Enum<ReplicateLevel>::NAME = "replication";
template <> const char* Enum<ReplicateLevel>::NAMES[] = { "none", "configuration", "all" };
template <> const size_t Enum<ReplicateLevel>::N = 3;
template <> const char* Enum<BrokerStatus>::NAME = "HA broker status";
// NOTE: Changing status names will have an impact on qpid-ha and
// the qpidd-primary init script.
// Don't change them unless you are going to update all dependent code.
//
template <> const char* Enum<BrokerStatus>::NAMES[] = {
"joining", "catchup", "ready", "recovering", "active", "standalone"
};
template <> const size_t Enum<BrokerStatus>::N = 6;
ostream& operator<<(ostream& o, EnumBase e) {
return o << e.str();
}
istream& operator>>(istream& i, EnumBase& e) {
string s;
i >> s;
e.parse(s);
return i;
}
ostream& operator<<(ostream& o, const UuidSet& ids) {
ostream_iterator<qpid::types::Uuid> out(o, " ");
o << "{ ";
for (UuidSet::const_iterator i = ids.begin(); i != ids.end(); ++i)
o << shortStr(*i) << " ";
o << "}";
return o;
}
std::string logMessageId(const std::string& q, QueuePosition pos, ReplicationId id) {
return Msg() << q << "[" << pos << "]" << "=" << id;
}
std::string logMessageId(const std::string& q, ReplicationId id) {
return Msg() << q << "[]" << "=" << id;
}
std::string logMessageId(const std::string& q, const broker::Message& m) {
return logMessageId(q, m.getSequence(), m.getReplicationId());
}
std::string logMessageId(const broker::Queue& q, QueuePosition pos, ReplicationId id) {
return logMessageId(q.getName(), pos, id);
}
std::string logMessageId(const broker::Queue& q, ReplicationId id) {
return logMessageId(q.getName(), id);
}
std::string logMessageId(const broker::Queue& q, const broker::Message& m) {
return logMessageId(q.getName(), m);
}
void UuidSet::encode(framing::Buffer& b) const {
b.putLong(size());
for (const_iterator i = begin(); i != end(); ++i)
b.putRawData(i->data(), i->size());
}
void UuidSet::decode(framing::Buffer& b) {
size_t n = b.getLong();
for ( ; n > 0; --n) {
types::Uuid id;
b.getRawData(const_cast<unsigned char*>(id.data()), id.size());
insert(id);
}
}
size_t UuidSet::encodedSize() const {
return sizeof(uint32_t) + size()*16;
}
}} // namespace qpid::ha
| {'content_hash': 'f21d21cc471937097f1fbe5958e3b91d', 'timestamp': '', 'source': 'github', 'line_count': 119, 'max_line_length': 91, 'avg_line_length': 27.88235294117647, 'alnum_prop': 0.6347197106690777, 'repo_name': 'mbroadst/debian-qpid-cpp', 'id': '3088661c954598de1fa21e93a4e568376e1b2305', 'size': '4131', 'binary': False, 'copies': '3', 'ref': 'refs/heads/trusty', 'path': 'src/qpid/ha/types.cpp', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Batchfile', 'bytes': '2126'}, {'name': 'C', 'bytes': '67620'}, {'name': 'C#', 'bytes': '121990'}, {'name': 'C++', 'bytes': '7291355'}, {'name': 'CMake', 'bytes': '169753'}, {'name': 'Cucumber', 'bytes': '15299'}, {'name': 'Emacs Lisp', 'bytes': '7379'}, {'name': 'HTML', 'bytes': '4773'}, {'name': 'Makefile', 'bytes': '1261'}, {'name': 'Perl', 'bytes': '86022'}, {'name': 'Perl6', 'bytes': '2703'}, {'name': 'PowerShell', 'bytes': '51728'}, {'name': 'Python', 'bytes': '1523448'}, {'name': 'Ruby', 'bytes': '305919'}, {'name': 'Shell', 'bytes': '128410'}]} |
package com.amazonaws.services.lambda.model;
import java.io.Serializable;
import com.amazonaws.AmazonWebServiceRequest;
/**
* Container for the parameters to the {@link com.amazonaws.services.lambda.AWSLambda#listEventSourceMappings(ListEventSourceMappingsRequest) ListEventSourceMappings operation}.
* <p>
* Returns a list of event source mappings you created using the
* <code>CreateEventSourceMapping</code> (see CreateEventSourceMapping),
* where you identify a stream as an event source. This list does not
* include Amazon S3 event sources.
* </p>
* <p>
* For each mapping, the API returns configuration information. You can
* optionally specify filters to retrieve specific event source mappings.
* </p>
* <p>
* This operation requires permission for the
* <code>lambda:ListEventSourceMappings</code> action.
* </p>
*
* @see com.amazonaws.services.lambda.AWSLambda#listEventSourceMappings(ListEventSourceMappingsRequest)
*/
public class ListEventSourceMappingsRequest extends AmazonWebServiceRequest implements Serializable, Cloneable {
/**
* The Amazon Resource Name (ARN) of the Amazon Kinesis stream.
* <p>
* <b>Constraints:</b><br/>
* <b>Pattern: </b>arn:aws:([a-zA-Z0-9\-])+:([a-z]{2}-[a-z]+-\d{1})?:(\d{12})?:(.*)<br/>
*/
private String eventSourceArn;
/**
* The name of the Lambda function. <p> You can specify an unqualified
* function name (for example, "Thumbnail") or you can specify Amazon
* Resource Name (ARN) of the function (for example,
* "arn:aws:lambda:us-west-2:account-id:function:ThumbNail"). AWS Lambda
* also allows you to specify only the account ID qualifier (for example,
* "account-id:Thumbnail"). Note that the length constraint applies only
* to the ARN. If you specify only the function name, it is limited to 64
* character in length.
* <p>
* <b>Constraints:</b><br/>
* <b>Length: </b>1 - 140<br/>
* <b>Pattern: </b>(arn:aws:lambda:)?([a-z]{2}-[a-z]+-\d{1}:)?(\d{12}:)?(function:)?([a-zA-Z0-9-_]+)(:(\$LATEST|[a-zA-Z0-9-_]+))?<br/>
*/
private String functionName;
/**
* Optional string. An opaque pagination token returned from a previous
* <code>ListEventSourceMappings</code> operation. If present, specifies
* to continue the list from where the returning call left off.
*/
private String marker;
/**
* Optional integer. Specifies the maximum number of event sources to
* return in response. This value must be greater than 0.
* <p>
* <b>Constraints:</b><br/>
* <b>Range: </b>1 - 10000<br/>
*/
private Integer maxItems;
/**
* The Amazon Resource Name (ARN) of the Amazon Kinesis stream.
* <p>
* <b>Constraints:</b><br/>
* <b>Pattern: </b>arn:aws:([a-zA-Z0-9\-])+:([a-z]{2}-[a-z]+-\d{1})?:(\d{12})?:(.*)<br/>
*
* @return The Amazon Resource Name (ARN) of the Amazon Kinesis stream.
*/
public String getEventSourceArn() {
return eventSourceArn;
}
/**
* The Amazon Resource Name (ARN) of the Amazon Kinesis stream.
* <p>
* <b>Constraints:</b><br/>
* <b>Pattern: </b>arn:aws:([a-zA-Z0-9\-])+:([a-z]{2}-[a-z]+-\d{1})?:(\d{12})?:(.*)<br/>
*
* @param eventSourceArn The Amazon Resource Name (ARN) of the Amazon Kinesis stream.
*/
public void setEventSourceArn(String eventSourceArn) {
this.eventSourceArn = eventSourceArn;
}
/**
* The Amazon Resource Name (ARN) of the Amazon Kinesis stream.
* <p>
* Returns a reference to this object so that method calls can be chained together.
* <p>
* <b>Constraints:</b><br/>
* <b>Pattern: </b>arn:aws:([a-zA-Z0-9\-])+:([a-z]{2}-[a-z]+-\d{1})?:(\d{12})?:(.*)<br/>
*
* @param eventSourceArn The Amazon Resource Name (ARN) of the Amazon Kinesis stream.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public ListEventSourceMappingsRequest withEventSourceArn(String eventSourceArn) {
this.eventSourceArn = eventSourceArn;
return this;
}
/**
* The name of the Lambda function. <p> You can specify an unqualified
* function name (for example, "Thumbnail") or you can specify Amazon
* Resource Name (ARN) of the function (for example,
* "arn:aws:lambda:us-west-2:account-id:function:ThumbNail"). AWS Lambda
* also allows you to specify only the account ID qualifier (for example,
* "account-id:Thumbnail"). Note that the length constraint applies only
* to the ARN. If you specify only the function name, it is limited to 64
* character in length.
* <p>
* <b>Constraints:</b><br/>
* <b>Length: </b>1 - 140<br/>
* <b>Pattern: </b>(arn:aws:lambda:)?([a-z]{2}-[a-z]+-\d{1}:)?(\d{12}:)?(function:)?([a-zA-Z0-9-_]+)(:(\$LATEST|[a-zA-Z0-9-_]+))?<br/>
*
* @return The name of the Lambda function. <p> You can specify an unqualified
* function name (for example, "Thumbnail") or you can specify Amazon
* Resource Name (ARN) of the function (for example,
* "arn:aws:lambda:us-west-2:account-id:function:ThumbNail"). AWS Lambda
* also allows you to specify only the account ID qualifier (for example,
* "account-id:Thumbnail"). Note that the length constraint applies only
* to the ARN. If you specify only the function name, it is limited to 64
* character in length.
*/
public String getFunctionName() {
return functionName;
}
/**
* The name of the Lambda function. <p> You can specify an unqualified
* function name (for example, "Thumbnail") or you can specify Amazon
* Resource Name (ARN) of the function (for example,
* "arn:aws:lambda:us-west-2:account-id:function:ThumbNail"). AWS Lambda
* also allows you to specify only the account ID qualifier (for example,
* "account-id:Thumbnail"). Note that the length constraint applies only
* to the ARN. If you specify only the function name, it is limited to 64
* character in length.
* <p>
* <b>Constraints:</b><br/>
* <b>Length: </b>1 - 140<br/>
* <b>Pattern: </b>(arn:aws:lambda:)?([a-z]{2}-[a-z]+-\d{1}:)?(\d{12}:)?(function:)?([a-zA-Z0-9-_]+)(:(\$LATEST|[a-zA-Z0-9-_]+))?<br/>
*
* @param functionName The name of the Lambda function. <p> You can specify an unqualified
* function name (for example, "Thumbnail") or you can specify Amazon
* Resource Name (ARN) of the function (for example,
* "arn:aws:lambda:us-west-2:account-id:function:ThumbNail"). AWS Lambda
* also allows you to specify only the account ID qualifier (for example,
* "account-id:Thumbnail"). Note that the length constraint applies only
* to the ARN. If you specify only the function name, it is limited to 64
* character in length.
*/
public void setFunctionName(String functionName) {
this.functionName = functionName;
}
/**
* The name of the Lambda function. <p> You can specify an unqualified
* function name (for example, "Thumbnail") or you can specify Amazon
* Resource Name (ARN) of the function (for example,
* "arn:aws:lambda:us-west-2:account-id:function:ThumbNail"). AWS Lambda
* also allows you to specify only the account ID qualifier (for example,
* "account-id:Thumbnail"). Note that the length constraint applies only
* to the ARN. If you specify only the function name, it is limited to 64
* character in length.
* <p>
* Returns a reference to this object so that method calls can be chained together.
* <p>
* <b>Constraints:</b><br/>
* <b>Length: </b>1 - 140<br/>
* <b>Pattern: </b>(arn:aws:lambda:)?([a-z]{2}-[a-z]+-\d{1}:)?(\d{12}:)?(function:)?([a-zA-Z0-9-_]+)(:(\$LATEST|[a-zA-Z0-9-_]+))?<br/>
*
* @param functionName The name of the Lambda function. <p> You can specify an unqualified
* function name (for example, "Thumbnail") or you can specify Amazon
* Resource Name (ARN) of the function (for example,
* "arn:aws:lambda:us-west-2:account-id:function:ThumbNail"). AWS Lambda
* also allows you to specify only the account ID qualifier (for example,
* "account-id:Thumbnail"). Note that the length constraint applies only
* to the ARN. If you specify only the function name, it is limited to 64
* character in length.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public ListEventSourceMappingsRequest withFunctionName(String functionName) {
this.functionName = functionName;
return this;
}
/**
* Optional string. An opaque pagination token returned from a previous
* <code>ListEventSourceMappings</code> operation. If present, specifies
* to continue the list from where the returning call left off.
*
* @return Optional string. An opaque pagination token returned from a previous
* <code>ListEventSourceMappings</code> operation. If present, specifies
* to continue the list from where the returning call left off.
*/
public String getMarker() {
return marker;
}
/**
* Optional string. An opaque pagination token returned from a previous
* <code>ListEventSourceMappings</code> operation. If present, specifies
* to continue the list from where the returning call left off.
*
* @param marker Optional string. An opaque pagination token returned from a previous
* <code>ListEventSourceMappings</code> operation. If present, specifies
* to continue the list from where the returning call left off.
*/
public void setMarker(String marker) {
this.marker = marker;
}
/**
* Optional string. An opaque pagination token returned from a previous
* <code>ListEventSourceMappings</code> operation. If present, specifies
* to continue the list from where the returning call left off.
* <p>
* Returns a reference to this object so that method calls can be chained together.
*
* @param marker Optional string. An opaque pagination token returned from a previous
* <code>ListEventSourceMappings</code> operation. If present, specifies
* to continue the list from where the returning call left off.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public ListEventSourceMappingsRequest withMarker(String marker) {
this.marker = marker;
return this;
}
/**
* Optional integer. Specifies the maximum number of event sources to
* return in response. This value must be greater than 0.
* <p>
* <b>Constraints:</b><br/>
* <b>Range: </b>1 - 10000<br/>
*
* @return Optional integer. Specifies the maximum number of event sources to
* return in response. This value must be greater than 0.
*/
public Integer getMaxItems() {
return maxItems;
}
/**
* Optional integer. Specifies the maximum number of event sources to
* return in response. This value must be greater than 0.
* <p>
* <b>Constraints:</b><br/>
* <b>Range: </b>1 - 10000<br/>
*
* @param maxItems Optional integer. Specifies the maximum number of event sources to
* return in response. This value must be greater than 0.
*/
public void setMaxItems(Integer maxItems) {
this.maxItems = maxItems;
}
/**
* Optional integer. Specifies the maximum number of event sources to
* return in response. This value must be greater than 0.
* <p>
* Returns a reference to this object so that method calls can be chained together.
* <p>
* <b>Constraints:</b><br/>
* <b>Range: </b>1 - 10000<br/>
*
* @param maxItems Optional integer. Specifies the maximum number of event sources to
* return in response. This value must be greater than 0.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public ListEventSourceMappingsRequest withMaxItems(Integer maxItems) {
this.maxItems = maxItems;
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getEventSourceArn() != null) sb.append("EventSourceArn: " + getEventSourceArn() + ",");
if (getFunctionName() != null) sb.append("FunctionName: " + getFunctionName() + ",");
if (getMarker() != null) sb.append("Marker: " + getMarker() + ",");
if (getMaxItems() != null) sb.append("MaxItems: " + getMaxItems() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getEventSourceArn() == null) ? 0 : getEventSourceArn().hashCode());
hashCode = prime * hashCode + ((getFunctionName() == null) ? 0 : getFunctionName().hashCode());
hashCode = prime * hashCode + ((getMarker() == null) ? 0 : getMarker().hashCode());
hashCode = prime * hashCode + ((getMaxItems() == null) ? 0 : getMaxItems().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof ListEventSourceMappingsRequest == false) return false;
ListEventSourceMappingsRequest other = (ListEventSourceMappingsRequest)obj;
if (other.getEventSourceArn() == null ^ this.getEventSourceArn() == null) return false;
if (other.getEventSourceArn() != null && other.getEventSourceArn().equals(this.getEventSourceArn()) == false) return false;
if (other.getFunctionName() == null ^ this.getFunctionName() == null) return false;
if (other.getFunctionName() != null && other.getFunctionName().equals(this.getFunctionName()) == false) return false;
if (other.getMarker() == null ^ this.getMarker() == null) return false;
if (other.getMarker() != null && other.getMarker().equals(this.getMarker()) == false) return false;
if (other.getMaxItems() == null ^ this.getMaxItems() == null) return false;
if (other.getMaxItems() != null && other.getMaxItems().equals(this.getMaxItems()) == false) return false;
return true;
}
@Override
public ListEventSourceMappingsRequest clone() {
return (ListEventSourceMappingsRequest) super.clone();
}
}
| {'content_hash': 'cb90ef3b857eb8c5397f5a3984299a48', 'timestamp': '', 'source': 'github', 'line_count': 349, 'max_line_length': 177, 'avg_line_length': 43.64756446991404, 'alnum_prop': 0.6281756712400709, 'repo_name': 'sdole/aws-sdk-java', 'id': 'b9a189e8fa04f19eb34f188672731c8eda1ff188', 'size': '15820', 'binary': False, 'copies': '2', 'ref': 'refs/heads/master', 'path': 'aws-java-sdk-lambda/src/main/java/com/amazonaws/services/lambda/model/ListEventSourceMappingsRequest.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Java', 'bytes': '97734456'}, {'name': 'Scilab', 'bytes': '2354'}]} |
/* */
var MapCache = require('./_MapCache');
var FUNC_ERROR_TEXT = 'Expected a function';
function memoize(func, resolver) {
if (typeof func != 'function' || (resolver != null && typeof resolver != 'function')) {
throw new TypeError(FUNC_ERROR_TEXT);
}
var memoized = function() {
var args = arguments,
key = resolver ? resolver.apply(this, args) : args[0],
cache = memoized.cache;
if (cache.has(key)) {
return cache.get(key);
}
var result = func.apply(this, args);
memoized.cache = cache.set(key, result) || cache;
return result;
};
memoized.cache = new (memoize.Cache || MapCache);
return memoized;
}
memoize.Cache = MapCache;
module.exports = memoize;
| {'content_hash': 'b944ad8be55e4358263ada0f450ec961', 'timestamp': '', 'source': 'github', 'line_count': 23, 'max_line_length': 89, 'avg_line_length': 31.217391304347824, 'alnum_prop': 0.6309192200557103, 'repo_name': 'onlabsorg/olowc', 'id': 'b51dbeabd4318c9550b9f3cfb116530a86677b74', 'size': '718', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'jspm_packages/npm/[email protected]/memoize.js', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'HTML', 'bytes': '2128'}, {'name': 'JavaScript', 'bytes': '2069038'}, {'name': 'Makefile', 'bytes': '188'}, {'name': 'OCaml', 'bytes': '485'}, {'name': 'Shell', 'bytes': '973'}]} |
using UnityEngine;
using System.Collections;
using System.Collections.Generic;
using System.Xml;
using System;
public class SystemScrollviews : MonoBehaviour
{
public GameObject improvementMessageLabel, availableImprovements, buttonLabel, improvementParent, improvementsWindow, improvementDetails;
public int techTierToShow, selectedPlanet, selectedSystem, selectedSlot;
public GameObject[] tabs = new GameObject[4];
private string improvementText, currentImprovement;
public UILabel improvementLabel, improvementWealthCost, improvementPowerCost, systemEffects, improvementWealthUpkeep, improvementPowerUpkeep, systemUpkeepPower, systemUpkeepWealth;
public GameObject[] unbuiltImprovementList = new GameObject[10];
public GameObject[] improvementsList = new GameObject[8];
private ImprovementsBasic improvementsBasic;
void Start()
{
SetUpImprovementLabels ();
selectedPlanet = -1;
}
private void SetUpImprovementLabels()
{
for(int i = 0; i < improvementsList.Length; ++i)
{
EventDelegate.Add(improvementsList[i].GetComponent<UIButton>().onClick, OpenImprovementsWindow);
NGUITools.SetActive(improvementsList[i], false); //Default set improvement to false so it won't be shown in scrollview unless needed
}
for(int i = 0; i < unbuiltImprovementList.Length; ++i)
{
NGUITools.SetActive(unbuiltImprovementList[i], false);
EventDelegate.Add(unbuiltImprovementList[i].GetComponent<UIButton>().onClick, ShowDetails);
}
}
private void OpenImprovementsWindow()
{
if(MasterScript.systemListConstructor.systemList[selectedSystem].systemOwnedBy == MasterScript.playerTurnScript.playerRace)
{
NGUITools.SetActive (improvementsWindow, true);
NGUITools.SetActive (improvementDetails, false);
currentImprovement = null;
bool reset = false;
for(int i = 0; i < tabs.Length; ++i)
{
if(tabs[i].GetComponent<UISprite>().spriteName == "Button Hover (Orange)")
{
UpdateImprovementsWindow (i);
reset = true;
break;
}
}
if(reset == false)
{
tabs[0].GetComponent<UIButton>().enabled = false;
tabs[0].GetComponent<UISprite>().spriteName = "Button Hover (Orange)";
UpdateImprovementsWindow (0);
}
selectedSlot = -1;
for(int i = 0; i < improvementsList.Length; ++i)
{
if(UIButton.current.gameObject == improvementsList[i])
{
selectedSlot = i;
break;
}
}
}
}
private void ShowDetails()
{
for(int i = 0; i < unbuiltImprovementList.Length; ++i)
{
if(UIButton.current.gameObject == unbuiltImprovementList[i])
{
unbuiltImprovementList[i].GetComponent<UIButton>().enabled = false;
unbuiltImprovementList[i].GetComponent<UISprite>().spriteName = "Button Hover (Orange)";
currentImprovement = UIButton.current.transform.Find ("Label").gameObject.GetComponent<UILabel>().text;
continue;
}
else
{
unbuiltImprovementList[i].GetComponent<UISprite>().spriteName = "Button Click";
unbuiltImprovementList[i].GetComponent<UIButton>().enabled = true;
}
}
Vector3 tempPos = UIButton.current.transform.localPosition;
improvementDetails.transform.localPosition = new Vector3 (tempPos.x + 265f, tempPos.y, tempPos.z);
for(int i = 0; i < MasterScript.systemListConstructor.basicImprovementsList.Count; ++i)
{
if(MasterScript.systemListConstructor.basicImprovementsList[i].name.ToUpper() == UIButton.current.transform.Find ("Label").GetComponent<UILabel>().text)
{
improvementLabel.text = MasterScript.systemListConstructor.basicImprovementsList[i].details;
improvementPowerCost.text = MasterScript.systemListConstructor.basicImprovementsList[i].cost.ToString();
improvementWealthCost.text = (MasterScript.systemListConstructor.basicImprovementsList[i].cost / 25).ToString();
improvementPowerUpkeep.text = "-" + MasterScript.systemListConstructor.basicImprovementsList[i].powerUpkeep.ToString();
improvementWealthUpkeep.text = "-" + MasterScript.systemListConstructor.basicImprovementsList[i].wealthUpkeep.ToString();
}
}
NGUITools.SetActive (improvementDetails, true);
}
private void UpdateImprovementsWindow(int level)
{
for(int i = 0; i < unbuiltImprovementList.Length; ++i)
{
NGUITools.SetActive(unbuiltImprovementList[i], false);
}
int j = 0;
for(int i = 0; i < improvementsBasic.listOfImprovements.Count; ++i)
{
if(improvementsBasic.listOfImprovements[i].improvementLevel == level)
{
if(improvementsBasic.listOfImprovements[i].improvementCategory == "Generic" || improvementsBasic.listOfImprovements[i].improvementCategory == "Defence"
|| improvementsBasic.listOfImprovements[i].improvementCategory == MasterScript.playerTurnScript.playerRace)
{
if(improvementsBasic.listOfImprovements[i].hasBeenBuilt == false)
{
NGUITools.SetActive(unbuiltImprovementList[j], true);
unbuiltImprovementList[j].transform.Find("Label").GetComponent<UILabel>().text = improvementsBasic.listOfImprovements[i].improvementName.ToUpper();
++j;
}
}
}
}
for(int i = j; j < unbuiltImprovementList.Length; ++j)
{
NGUITools.SetActive(unbuiltImprovementList[i], false);
}
}
public void UpdateBuiltImprovements()
{
for(int i = 0; i < improvementsList.Length; ++i) //For all improvement slots
{
if(i < MasterScript.systemListConstructor.systemList[selectedSystem].planetsInSystem[selectedPlanet].currentImprovementSlots) //If is equal to or less than planets slots
{
NGUITools.SetActive(improvementsList[i], true); //Activate
if(MasterScript.systemListConstructor.systemList[selectedSystem].planetsInSystem[selectedPlanet].improvementsBuilt[i] != null) //If something built
{
improvementsList[i].transform.Find ("Name").GetComponent<UILabel>().text = MasterScript.systemListConstructor.systemList[selectedSystem].planetsInSystem[selectedPlanet].improvementsBuilt[i].ToUpper(); //Set text
improvementsList[i].GetComponent<UIButton>().enabled = false;
improvementsList[i].GetComponent<UISprite>().spriteName = "Button Normal";
}
else //Else say is empty
{
improvementsList[i].transform.Find ("Name").GetComponent<UILabel>().text = "Empty";
if(selectedSlot == i)
{
improvementsList[i].GetComponent<UIButton>().enabled = false;
improvementsList[i].GetComponent<UISprite>().spriteName = "Button Hover (Orange)";
}
else
{
improvementsList[i].GetComponent<UIButton>().enabled = true;
}
}
continue;
}
else //Else deactivate
{
NGUITools.SetActive(improvementsList[i], false);
}
}
}
private void UpdateTabs()
{
for(int i = 0; i < tabs.Length; ++i)
{
if(i <= improvementsBasic.techTier)
{
if(tabs[i].GetComponent<UISprite>().spriteName == "Button Hover (Orange)")
{
continue;
}
else
{
tabs[i].GetComponent<UIButton>().enabled = true;
tabs[i].GetComponent<UISprite>().spriteName = "Button Normal";
}
}
else
{
tabs[i].GetComponent<UIButton>().enabled = false;
tabs[i].GetComponent<UISprite>().spriteName = "Button Deactivated";
}
}
}
public void TabClick()
{
NGUITools.SetActive (improvementDetails, false);
currentImprovement = null;
for(int i = 0; i < tabs.Length; ++i)
{
if(tabs[i] == UIButton.current.gameObject)
{
tabs[i].GetComponent<UIButton>().enabled = false;
tabs[i].GetComponent<UISprite>().spriteName = "Button Hover (Orange)";
UpdateImprovementsWindow(i);
}
else
{
if(i <= improvementsBasic.techTier)
{
tabs[i].GetComponent<UIButton>().enabled = true;
tabs[i].GetComponent<UISprite>().spriteName = "Button Normal";
}
else
{
tabs[i].GetComponent<UIButton>().enabled = false;
tabs[i].GetComponent<UISprite>().spriteName = "Button Deactivated";
}
}
}
}
private void UpdateUpkeep()
{
float upkeepWealth = 0, upkeepPower = 0;
for(int i = 0; i < MasterScript.systemListConstructor.systemList[selectedSystem].planetsInSystem[selectedPlanet].improvementsBuilt.Count; ++i)
{
for(int j = 0; j < MasterScript.systemListConstructor.basicImprovementsList.Count; ++j)
{
if(improvementsBasic.listOfImprovements[j].hasBeenBuilt == false)
{
continue;
}
if(MasterScript.systemListConstructor.systemList[selectedSystem].planetsInSystem[selectedPlanet].improvementsBuilt[i] == MasterScript.systemListConstructor.basicImprovementsList[j].name)
{
upkeepWealth += MasterScript.systemListConstructor.basicImprovementsList[j].wealthUpkeep;
upkeepPower += MasterScript.systemListConstructor.basicImprovementsList[j].powerUpkeep;
continue;
}
}
}
systemUpkeepPower.text = upkeepPower.ToString();
systemUpkeepWealth.text = upkeepWealth.ToString ();
}
void Update()
{
if(MasterScript.systemGUI.selectedSystem != selectedSystem)
{
NGUITools.SetActive(improvementsWindow, false);
selectedSystem = MasterScript.systemGUI.selectedSystem;
improvementsBasic = MasterScript.systemListConstructor.systemList [selectedSystem].systemObject.GetComponent<ImprovementsBasic> ();
}
if(MasterScript.cameraFunctionsScript.openMenu == true)
{
if(selectedPlanet != -1)
{
if(improvementsWindow.activeInHierarchy == true)
{
UpdateTabs();
}
UpdateBuiltImprovements();
UpdateSystemEffects (selectedSystem, selectedPlanet);
UpdateUpkeep();
}
}
if(Input.GetKeyDown("c"))
{
NGUITools.SetActive(availableImprovements, false);
}
}
public void UpdateSystemEffects(int system, int planet) //TODO this needs to be planet specific
{
improvementsBasic = MasterScript.systemListConstructor.systemList[selectedSystem].systemObject.GetComponent<ImprovementsBasic>();
string temp = "";
float knoTemp = (MasterScript.systemListConstructor.systemList[system].sysKnowledgeModifier + MasterScript.systemListConstructor.systemList[system].planetsInSystem[planet].knowledgeModifier) - 1;
float powTemp = (MasterScript.systemListConstructor.systemList[system].sysPowerModifier + MasterScript.systemListConstructor.systemList[system].planetsInSystem[planet].powerModifier) - 1;
float growTemp = MasterScript.systemListConstructor.systemList[system].sysGrowthModifier + MasterScript.systemListConstructor.systemList[system].planetsInSystem[planet].growthModifier;
float popTemp = MasterScript.systemListConstructor.systemList[system].sysMaxPopulationModifier + MasterScript.systemListConstructor.systemList[system].planetsInSystem[planet].maxPopulationModifier;
float amberPenalty = MasterScript.systemListConstructor.systemList[system].sysAmberPenalty + MasterScript.systemListConstructor.systemList[system].planetsInSystem[planet].amberPenalty;
float amberProd = (MasterScript.systemListConstructor.systemList[system].sysAmberModifier + MasterScript.systemListConstructor.systemList[system].planetsInSystem[planet].amberModifier) - 1;
if(knoTemp != 0f)
{
if(temp != "")
{
temp = temp + "\n+";
}
temp = temp + Math.Round(knoTemp * 100, 1) + "% Knowledge from Improvements";
}
if(powTemp != 0f)
{
if(temp != "")
{
temp = temp + "\n+";
}
temp = temp + Math.Round(powTemp * 100, 1) + "% Power from Improvements";
}
if(growTemp != 0f)
{
if(temp != "")
{
temp = temp + "\n+";
}
temp = temp + Math.Round(growTemp, 2) + "% Growth from Improvements";
}
if(popTemp != 0f)
{
if(temp != "")
{
temp = temp + "\n+";
}
temp = temp + Math.Round(popTemp, 1) + "% Population from Improvements";
}
int standardSize = MasterScript.systemListConstructor.systemList[selectedSystem].planetsInSystem[selectedPlanet].baseImprovementSlots;
if(MasterScript.systemListConstructor.systemList[selectedSystem].planetsInSystem[selectedPlanet].currentImprovementSlots > standardSize)
{
if(temp != "")
{
temp = temp + "\n+";
}
temp = temp + (MasterScript.systemListConstructor.systemList[selectedSystem].planetsInSystem[selectedPlanet].currentImprovementSlots - standardSize).ToString() + " Improvement Slots on Planet";
}
if(amberPenalty != 1f)
{
if(temp != "")
{
temp = temp + "\n+";
}
temp = temp + Math.Round ((1 - amberPenalty) * 100, 1) + "% Amber Penalty on System";
}
if(amberProd != 0)
{
if(temp != "")
{
temp = temp + "\n+";
}
temp = temp + Math.Round (amberProd * 100, 1) + "% Amber Production on System";
}
if(improvementsBasic.improvementCostModifier != 0f)
{
if(temp != "")
{
temp = temp + "\n";
}
temp = temp + improvementsBasic.improvementCostModifier + " less Power required for Improvements";
}
if(improvementsBasic.researchCost != 0f)
{
if(temp != "")
{
temp = temp + "\n";
}
temp = temp + improvementsBasic.researchCost + " less Knowledge required for Research";
}
/*
amberPointBonus;
public float tempWealth, tempKnwlUnitBonus, tempPowUnitBonus, tempResearchCostReduction, tempImprovementCostReduction,
tempBonusAmbition;
for(int i = 0; i < improvementsBasic.listOfImprovements.Count; ++i)
{
if(improvementsBasic.listOfImprovements[i].hasBeenBuilt == true)
{
if(temp == "")
{
temp = improvementsBasic.listOfImprovements[i].improvementMessage.ToUpper();
}
else
{
temp = temp + "\n" + improvementsBasic.listOfImprovements[i].improvementMessage.ToUpper();
}
}
}
*/
if(temp == "")
{
temp = "NO EFFECTS ON SYSTEM";
}
systemEffects.text = temp;
systemEffects.transform.parent.GetComponent<UISprite> ().height = systemEffects.height + 20;
}
public void BuildImprovement()
{
NGUITools.SetActive (improvementDetails, false);
improvementsBasic = MasterScript.systemListConstructor.systemList[selectedSystem].systemObject.GetComponent<ImprovementsBasic>();
for(int i = 0; i < improvementsBasic.listOfImprovements.Count; ++i)
{
if(improvementsBasic.listOfImprovements[i].improvementName.ToUpper () == currentImprovement)
{
for(int j = 0; j < MasterScript.systemListConstructor.systemList[selectedSystem].planetsInSystem[selectedPlanet].currentImprovementSlots; ++j)
{
if(MasterScript.systemListConstructor.systemList[selectedSystem].planetsInSystem[selectedPlanet].improvementsBuilt[j] == null)
{
if(improvementsBasic.ImproveSystem(i) == true)
{
improvementsBasic.ActiveTechnologies(selectedSystem, MasterScript.playerTurnScript);
MasterScript.systemListConstructor.systemList[selectedSystem].planetsInSystem[selectedPlanet].improvementsBuilt[j] = improvementsBasic.listOfImprovements[i].improvementName;
UpdateImprovementsWindow(improvementsBasic.listOfImprovements[i].improvementLevel);
UpdateBuiltImprovements();
currentImprovement = null;
selectedSlot = -1;
break;
}
}
}
}
}
for(int i = 0; i < unbuiltImprovementList.Length; ++i)
{
unbuiltImprovementList[i].GetComponent<UISprite>().spriteName = "Button Normal";
unbuiltImprovementList[i].GetComponent<UIButton>().enabled = true;
}
NGUITools.SetActive(improvementsWindow, false);
}
private void CheckForTierUnlock()
{
for(int i = 0; i < 4; ++i)
{
UIButton temp = tabs[i].gameObject.GetComponent<UIButton>();
if(improvementsBasic.techTier >= i && temp.enabled == false)
{
temp.enabled = true;
}
if(improvementsBasic.techTier < i && temp.enabled == true)
{
temp.enabled = false;
}
}
}
}
| {'content_hash': '6c52717d8bbbbb1ae9c235432a053de8', 'timestamp': '', 'source': 'github', 'line_count': 490, 'max_line_length': 216, 'avg_line_length': 31.63469387755102, 'alnum_prop': 0.7123411392813367, 'repo_name': 'Shemamforash/Crucible', 'id': '84fa05cdf43143fdf158fe3ded82afdb878c135b', 'size': '15503', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'Scripts/UIScripts/SystemScrollviews.cs', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'C#', 'bytes': '376581'}]} |
r"""HTTP cookie handling for web clients.
This module has (now fairly distant) origins in Gisle Aas' Perl module
HTTP::Cookies, from the libwww-perl library.
Docstrings, comments and debug strings in this code refer to the
attributes of the HTTP cookie system as cookie-attributes, to distinguish
them clearly from Python attributes.
Class diagram (note that BSDDBCookieJar and the MSIE* classes are not
distributed with the Python standard library, but are available from
http://wwwsearch.sf.net/):
CookieJar____
/ \ \
FileCookieJar \ \
/ | \ \ \
MozillaCookieJar | LWPCookieJar \ \
| | \
| ---MSIEBase | \
| / | | \
| / MSIEDBCookieJar BSDDBCookieJar
|/
MSIECookieJar
"""
__all__ = ['Cookie', 'CookieJar', 'CookiePolicy', 'DefaultCookiePolicy',
'FileCookieJar', 'LWPCookieJar', 'LoadError', 'MozillaCookieJar']
import copy
import datetime
import re
import time
import urllib.parse, urllib.request
try:
import threading as _threading
except ImportError:
import dummy_threading as _threading
import http.client # only for the default HTTP port
from calendar import timegm
debug = False # set to True to enable debugging via the logging module
logger = None
def _debug(*args):
if not debug:
return
global logger
if not logger:
import logging
logger = logging.getLogger("http.cookiejar")
return logger.debug(*args)
DEFAULT_HTTP_PORT = str(http.client.HTTP_PORT)
MISSING_FILENAME_TEXT = ("a filename was not supplied (nor was the CookieJar "
"instance initialised with one)")
def _warn_unhandled_exception():
# There are a few catch-all except: statements in this module, for
# catching input that's bad in unexpected ways. Warn if any
# exceptions are caught there.
import io, warnings, traceback
f = io.StringIO()
traceback.print_exc(None, f)
msg = f.getvalue()
warnings.warn("http.cookiejar bug!\n%s" % msg, stacklevel=2)
# Date/time conversion
# -----------------------------------------------------------------------------
EPOCH_YEAR = 1970
def _timegm(tt):
year, month, mday, hour, min, sec = tt[:6]
if ((year >= EPOCH_YEAR) and (1 <= month <= 12) and (1 <= mday <= 31) and
(0 <= hour <= 24) and (0 <= min <= 59) and (0 <= sec <= 61)):
return timegm(tt)
else:
return None
DAYS = ["Mon", "Tue", "Wed", "Thu", "Fri", "Sat", "Sun"]
MONTHS = ["Jan", "Feb", "Mar", "Apr", "May", "Jun",
"Jul", "Aug", "Sep", "Oct", "Nov", "Dec"]
MONTHS_LOWER = []
for month in MONTHS: MONTHS_LOWER.append(month.lower())
def time2isoz(t=None):
"""Return a string representing time in seconds since epoch, t.
If the function is called without an argument, it will use the current
time.
The format of the returned string is like "YYYY-MM-DD hh:mm:ssZ",
representing Universal Time (UTC, aka GMT). An example of this format is:
1994-11-24 08:49:37Z
"""
if t is None:
dt = datetime.datetime.utcnow()
else:
dt = datetime.datetime.utcfromtimestamp(t)
return "%04d-%02d-%02d %02d:%02d:%02dZ" % (
dt.year, dt.month, dt.day, dt.hour, dt.minute, dt.second)
def time2netscape(t=None):
"""Return a string representing time in seconds since epoch, t.
If the function is called without an argument, it will use the current
time.
The format of the returned string is like this:
Wed, DD-Mon-YYYY HH:MM:SS GMT
"""
if t is None:
dt = datetime.datetime.utcnow()
else:
dt = datetime.datetime.utcfromtimestamp(t)
return "%s %02d-%s-%04d %02d:%02d:%02d GMT" % (
DAYS[dt.weekday()], dt.day, MONTHS[dt.month-1],
dt.year, dt.hour, dt.minute, dt.second)
UTC_ZONES = {"GMT": None, "UTC": None, "UT": None, "Z": None}
TIMEZONE_RE = re.compile(r"^([-+])?(\d\d?):?(\d\d)?$", re.ASCII)
def offset_from_tz_string(tz):
offset = None
if tz in UTC_ZONES:
offset = 0
else:
m = TIMEZONE_RE.search(tz)
if m:
offset = 3600 * int(m.group(2))
if m.group(3):
offset = offset + 60 * int(m.group(3))
if m.group(1) == '-':
offset = -offset
return offset
def _str2time(day, mon, yr, hr, min, sec, tz):
# translate month name to number
# month numbers start with 1 (January)
try:
mon = MONTHS_LOWER.index(mon.lower())+1
except ValueError:
# maybe it's already a number
try:
imon = int(mon)
except ValueError:
return None
if 1 <= imon <= 12:
mon = imon
else:
return None
# make sure clock elements are defined
if hr is None: hr = 0
if min is None: min = 0
if sec is None: sec = 0
yr = int(yr)
day = int(day)
hr = int(hr)
min = int(min)
sec = int(sec)
if yr < 1000:
# find "obvious" year
cur_yr = time.localtime(time.time())[0]
m = cur_yr % 100
tmp = yr
yr = yr + cur_yr - m
m = m - tmp
if abs(m) > 50:
if m > 0: yr = yr + 100
else: yr = yr - 100
# convert UTC time tuple to seconds since epoch (not timezone-adjusted)
t = _timegm((yr, mon, day, hr, min, sec, tz))
if t is not None:
# adjust time using timezone string, to get absolute time since epoch
if tz is None:
tz = "UTC"
tz = tz.upper()
offset = offset_from_tz_string(tz)
if offset is None:
return None
t = t - offset
return t
STRICT_DATE_RE = re.compile(
r"^[SMTWF][a-z][a-z], (\d\d) ([JFMASOND][a-z][a-z]) "
"(\d\d\d\d) (\d\d):(\d\d):(\d\d) GMT$", re.ASCII)
WEEKDAY_RE = re.compile(
r"^(?:Sun|Mon|Tue|Wed|Thu|Fri|Sat)[a-z]*,?\s*", re.I | re.ASCII)
LOOSE_HTTP_DATE_RE = re.compile(
r"""^
(\d\d?) # day
(?:\s+|[-\/])
(\w+) # month
(?:\s+|[-\/])
(\d+) # year
(?:
(?:\s+|:) # separator before clock
(\d\d?):(\d\d) # hour:min
(?::(\d\d))? # optional seconds
)? # optional clock
\s*
([-+]?\d{2,4}|(?![APap][Mm]\b)[A-Za-z]+)? # timezone
\s*
(?:\(\w+\))? # ASCII representation of timezone in parens.
\s*$""", re.X | re.ASCII)
def http2time(text):
"""Returns time in seconds since epoch of time represented by a string.
Return value is an integer.
None is returned if the format of str is unrecognized, the time is outside
the representable range, or the timezone string is not recognized. If the
string contains no timezone, UTC is assumed.
The timezone in the string may be numerical (like "-0800" or "+0100") or a
string timezone (like "UTC", "GMT", "BST" or "EST"). Currently, only the
timezone strings equivalent to UTC (zero offset) are known to the function.
The function loosely parses the following formats:
Wed, 09 Feb 1994 22:23:32 GMT -- HTTP format
Tuesday, 08-Feb-94 14:15:29 GMT -- old rfc850 HTTP format
Tuesday, 08-Feb-1994 14:15:29 GMT -- broken rfc850 HTTP format
09 Feb 1994 22:23:32 GMT -- HTTP format (no weekday)
08-Feb-94 14:15:29 GMT -- rfc850 format (no weekday)
08-Feb-1994 14:15:29 GMT -- broken rfc850 format (no weekday)
The parser ignores leading and trailing whitespace. The time may be
absent.
If the year is given with only 2 digits, the function will select the
century that makes the year closest to the current date.
"""
# fast exit for strictly conforming string
m = STRICT_DATE_RE.search(text)
if m:
g = m.groups()
mon = MONTHS_LOWER.index(g[1].lower()) + 1
tt = (int(g[2]), mon, int(g[0]),
int(g[3]), int(g[4]), float(g[5]))
return _timegm(tt)
# No, we need some messy parsing...
# clean up
text = text.lstrip()
text = WEEKDAY_RE.sub("", text, 1) # Useless weekday
# tz is time zone specifier string
day, mon, yr, hr, min, sec, tz = [None]*7
# loose regexp parse
m = LOOSE_HTTP_DATE_RE.search(text)
if m is not None:
day, mon, yr, hr, min, sec, tz = m.groups()
else:
return None # bad format
return _str2time(day, mon, yr, hr, min, sec, tz)
ISO_DATE_RE = re.compile(
"""^
(\d{4}) # year
[-\/]?
(\d\d?) # numerical month
[-\/]?
(\d\d?) # day
(?:
(?:\s+|[-:Tt]) # separator before clock
(\d\d?):?(\d\d) # hour:min
(?::?(\d\d(?:\.\d*)?))? # optional seconds (and fractional)
)? # optional clock
\s*
([-+]?\d\d?:?(:?\d\d)?
|Z|z)? # timezone (Z is "zero meridian", i.e. GMT)
\s*$""", re.X | re. ASCII)
def iso2time(text):
"""
As for http2time, but parses the ISO 8601 formats:
1994-02-03 14:15:29 -0100 -- ISO 8601 format
1994-02-03 14:15:29 -- zone is optional
1994-02-03 -- only date
1994-02-03T14:15:29 -- Use T as separator
19940203T141529Z -- ISO 8601 compact format
19940203 -- only date
"""
# clean up
text = text.lstrip()
# tz is time zone specifier string
day, mon, yr, hr, min, sec, tz = [None]*7
# loose regexp parse
m = ISO_DATE_RE.search(text)
if m is not None:
# XXX there's an extra bit of the timezone I'm ignoring here: is
# this the right thing to do?
yr, mon, day, hr, min, sec, tz, _ = m.groups()
else:
return None # bad format
return _str2time(day, mon, yr, hr, min, sec, tz)
# Header parsing
# -----------------------------------------------------------------------------
def unmatched(match):
"""Return unmatched part of re.Match object."""
start, end = match.span(0)
return match.string[:start]+match.string[end:]
HEADER_TOKEN_RE = re.compile(r"^\s*([^=\s;,]+)")
HEADER_QUOTED_VALUE_RE = re.compile(r"^\s*=\s*\"([^\"\\]*(?:\\.[^\"\\]*)*)\"")
HEADER_VALUE_RE = re.compile(r"^\s*=\s*([^\s;,]*)")
HEADER_ESCAPE_RE = re.compile(r"\\(.)")
def split_header_words(header_values):
r"""Parse header values into a list of lists containing key,value pairs.
The function knows how to deal with ",", ";" and "=" as well as quoted
values after "=". A list of space separated tokens are parsed as if they
were separated by ";".
If the header_values passed as argument contains multiple values, then they
are treated as if they were a single value separated by comma ",".
This means that this function is useful for parsing header fields that
follow this syntax (BNF as from the HTTP/1.1 specification, but we relax
the requirement for tokens).
headers = #header
header = (token | parameter) *( [";"] (token | parameter))
token = 1*<any CHAR except CTLs or separators>
separators = "(" | ")" | "<" | ">" | "@"
| "," | ";" | ":" | "\" | <">
| "/" | "[" | "]" | "?" | "="
| "{" | "}" | SP | HT
quoted-string = ( <"> *(qdtext | quoted-pair ) <"> )
qdtext = <any TEXT except <">>
quoted-pair = "\" CHAR
parameter = attribute "=" value
attribute = token
value = token | quoted-string
Each header is represented by a list of key/value pairs. The value for a
simple token (not part of a parameter) is None. Syntactically incorrect
headers will not necessarily be parsed as you would want.
This is easier to describe with some examples:
>>> split_header_words(['foo="bar"; port="80,81"; discard, bar=baz'])
[[('foo', 'bar'), ('port', '80,81'), ('discard', None)], [('bar', 'baz')]]
>>> split_header_words(['text/html; charset="iso-8859-1"'])
[[('text/html', None), ('charset', 'iso-8859-1')]]
>>> split_header_words([r'Basic realm="\"foo\bar\""'])
[[('Basic', None), ('realm', '"foobar"')]]
"""
assert not isinstance(header_values, str)
result = []
for text in header_values:
orig_text = text
pairs = []
while text:
m = HEADER_TOKEN_RE.search(text)
if m:
text = unmatched(m)
name = m.group(1)
m = HEADER_QUOTED_VALUE_RE.search(text)
if m: # quoted value
text = unmatched(m)
value = m.group(1)
value = HEADER_ESCAPE_RE.sub(r"\1", value)
else:
m = HEADER_VALUE_RE.search(text)
if m: # unquoted value
text = unmatched(m)
value = m.group(1)
value = value.rstrip()
else:
# no value, a lone token
value = None
pairs.append((name, value))
elif text.lstrip().startswith(","):
# concatenated headers, as per RFC 2616 section 4.2
text = text.lstrip()[1:]
if pairs: result.append(pairs)
pairs = []
else:
# skip junk
non_junk, nr_junk_chars = re.subn("^[=\s;]*", "", text)
assert nr_junk_chars > 0, (
"split_header_words bug: '%s', '%s', %s" %
(orig_text, text, pairs))
text = non_junk
if pairs: result.append(pairs)
return result
HEADER_JOIN_ESCAPE_RE = re.compile(r"([\"\\])")
def join_header_words(lists):
"""Do the inverse (almost) of the conversion done by split_header_words.
Takes a list of lists of (key, value) pairs and produces a single header
value. Attribute values are quoted if needed.
>>> join_header_words([[("text/plain", None), ("charset", "iso-8859/1")]])
'text/plain; charset="iso-8859/1"'
>>> join_header_words([[("text/plain", None)], [("charset", "iso-8859/1")]])
'text/plain, charset="iso-8859/1"'
"""
headers = []
for pairs in lists:
attr = []
for k, v in pairs:
if v is not None:
if not re.search(r"^\w+$", v):
v = HEADER_JOIN_ESCAPE_RE.sub(r"\\\1", v) # escape " and \
v = '"%s"' % v
k = "%s=%s" % (k, v)
attr.append(k)
if attr: headers.append("; ".join(attr))
return ", ".join(headers)
def strip_quotes(text):
if text.startswith('"'):
text = text[1:]
if text.endswith('"'):
text = text[:-1]
return text
def parse_ns_headers(ns_headers):
"""Ad-hoc parser for Netscape protocol cookie-attributes.
The old Netscape cookie format for Set-Cookie can for instance contain
an unquoted "," in the expires field, so we have to use this ad-hoc
parser instead of split_header_words.
XXX This may not make the best possible effort to parse all the crap
that Netscape Cookie headers contain. Ronald Tschalar's HTTPClient
parser is probably better, so could do worse than following that if
this ever gives any trouble.
Currently, this is also used for parsing RFC 2109 cookies.
"""
known_attrs = ("expires", "domain", "path", "secure",
# RFC 2109 attrs (may turn up in Netscape cookies, too)
"version", "port", "max-age")
result = []
for ns_header in ns_headers:
pairs = []
version_set = False
for ii, param in enumerate(re.split(r";\s*", ns_header)):
param = param.rstrip()
if param == "": continue
if "=" not in param:
k, v = param, None
else:
k, v = re.split(r"\s*=\s*", param, 1)
k = k.lstrip()
if ii != 0:
lc = k.lower()
if lc in known_attrs:
k = lc
if k == "version":
# This is an RFC 2109 cookie.
v = strip_quotes(v)
version_set = True
if k == "expires":
# convert expires date to seconds since epoch
v = http2time(strip_quotes(v)) # None if invalid
pairs.append((k, v))
if pairs:
if not version_set:
pairs.append(("version", "0"))
result.append(pairs)
return result
IPV4_RE = re.compile(r"\.\d+$", re.ASCII)
def is_HDN(text):
"""Return True if text is a host domain name."""
# XXX
# This may well be wrong. Which RFC is HDN defined in, if any (for
# the purposes of RFC 2965)?
# For the current implementation, what about IPv6? Remember to look
# at other uses of IPV4_RE also, if change this.
if IPV4_RE.search(text):
return False
if text == "":
return False
if text[0] == "." or text[-1] == ".":
return False
return True
def domain_match(A, B):
"""Return True if domain A domain-matches domain B, according to RFC 2965.
A and B may be host domain names or IP addresses.
RFC 2965, section 1:
Host names can be specified either as an IP address or a HDN string.
Sometimes we compare one host name with another. (Such comparisons SHALL
be case-insensitive.) Host A's name domain-matches host B's if
* their host name strings string-compare equal; or
* A is a HDN string and has the form NB, where N is a non-empty
name string, B has the form .B', and B' is a HDN string. (So,
x.y.com domain-matches .Y.com but not Y.com.)
Note that domain-match is not a commutative operation: a.b.c.com
domain-matches .c.com, but not the reverse.
"""
# Note that, if A or B are IP addresses, the only relevant part of the
# definition of the domain-match algorithm is the direct string-compare.
A = A.lower()
B = B.lower()
if A == B:
return True
if not is_HDN(A):
return False
i = A.rfind(B)
if i == -1 or i == 0:
# A does not have form NB, or N is the empty string
return False
if not B.startswith("."):
return False
if not is_HDN(B[1:]):
return False
return True
def liberal_is_HDN(text):
"""Return True if text is a sort-of-like a host domain name.
For accepting/blocking domains.
"""
if IPV4_RE.search(text):
return False
return True
def user_domain_match(A, B):
"""For blocking/accepting domains.
A and B may be host domain names or IP addresses.
"""
A = A.lower()
B = B.lower()
if not (liberal_is_HDN(A) and liberal_is_HDN(B)):
if A == B:
# equal IP addresses
return True
return False
initial_dot = B.startswith(".")
if initial_dot and A.endswith(B):
return True
if not initial_dot and A == B:
return True
return False
cut_port_re = re.compile(r":\d+$", re.ASCII)
def request_host(request):
"""Return request-host, as defined by RFC 2965.
Variation from RFC: returned value is lowercased, for convenient
comparison.
"""
url = request.get_full_url()
host = urllib.parse.urlparse(url)[1]
if host == "":
host = request.get_header("Host", "")
# remove port, if present
host = cut_port_re.sub("", host, 1)
return host.lower()
def eff_request_host(request):
"""Return a tuple (request-host, effective request-host name).
As defined by RFC 2965, except both are lowercased.
"""
erhn = req_host = request_host(request)
if req_host.find(".") == -1 and not IPV4_RE.search(req_host):
erhn = req_host + ".local"
return req_host, erhn
def request_path(request):
"""Path component of request-URI, as defined by RFC 2965."""
url = request.get_full_url()
parts = urllib.parse.urlsplit(url)
path = escape_path(parts.path)
if not path.startswith("/"):
# fix bad RFC 2396 absoluteURI
path = "/" + path
return path
def request_port(request):
host = request.host
i = host.find(':')
if i >= 0:
port = host[i+1:]
try:
int(port)
except ValueError:
_debug("nonnumeric port: '%s'", port)
return None
else:
port = DEFAULT_HTTP_PORT
return port
# Characters in addition to A-Z, a-z, 0-9, '_', '.', and '-' that don't
# need to be escaped to form a valid HTTP URL (RFCs 2396 and 1738).
HTTP_PATH_SAFE = "%/;:@&=+$,!~*'()"
ESCAPED_CHAR_RE = re.compile(r"%([0-9a-fA-F][0-9a-fA-F])")
def uppercase_escaped_char(match):
return "%%%s" % match.group(1).upper()
def escape_path(path):
"""Escape any invalid characters in HTTP URL, and uppercase all escapes."""
# There's no knowing what character encoding was used to create URLs
# containing %-escapes, but since we have to pick one to escape invalid
# path characters, we pick UTF-8, as recommended in the HTML 4.0
# specification:
# http://www.w3.org/TR/REC-html40/appendix/notes.html#h-B.2.1
# And here, kind of: draft-fielding-uri-rfc2396bis-03
# (And in draft IRI specification: draft-duerst-iri-05)
# (And here, for new URI schemes: RFC 2718)
path = urllib.parse.quote(path, HTTP_PATH_SAFE)
path = ESCAPED_CHAR_RE.sub(uppercase_escaped_char, path)
return path
def reach(h):
"""Return reach of host h, as defined by RFC 2965, section 1.
The reach R of a host name H is defined as follows:
* If
- H is the host domain name of a host; and,
- H has the form A.B; and
- A has no embedded (that is, interior) dots; and
- B has at least one embedded dot, or B is the string "local".
then the reach of H is .B.
* Otherwise, the reach of H is H.
>>> reach("www.acme.com")
'.acme.com'
>>> reach("acme.com")
'acme.com'
>>> reach("acme.local")
'.local'
"""
i = h.find(".")
if i >= 0:
#a = h[:i] # this line is only here to show what a is
b = h[i+1:]
i = b.find(".")
if is_HDN(h) and (i >= 0 or b == "local"):
return "."+b
return h
def is_third_party(request):
"""
RFC 2965, section 3.3.6:
An unverifiable transaction is to a third-party host if its request-
host U does not domain-match the reach R of the request-host O in the
origin transaction.
"""
req_host = request_host(request)
if not domain_match(req_host, reach(request.origin_req_host)):
return True
else:
return False
class Cookie:
"""HTTP Cookie.
This class represents both Netscape and RFC 2965 cookies.
This is deliberately a very simple class. It just holds attributes. It's
possible to construct Cookie instances that don't comply with the cookie
standards. CookieJar.make_cookies is the factory function for Cookie
objects -- it deals with cookie parsing, supplying defaults, and
normalising to the representation used in this class. CookiePolicy is
responsible for checking them to see whether they should be accepted from
and returned to the server.
Note that the port may be present in the headers, but unspecified ("Port"
rather than"Port=80", for example); if this is the case, port is None.
"""
def __init__(self, version, name, value,
port, port_specified,
domain, domain_specified, domain_initial_dot,
path, path_specified,
secure,
expires,
discard,
comment,
comment_url,
rest,
rfc2109=False,
):
if version is not None: version = int(version)
if expires is not None: expires = int(expires)
if port is None and port_specified is True:
raise ValueError("if port is None, port_specified must be false")
self.version = version
self.name = name
self.value = value
self.port = port
self.port_specified = port_specified
# normalise case, as per RFC 2965 section 3.3.3
self.domain = domain.lower()
self.domain_specified = domain_specified
# Sigh. We need to know whether the domain given in the
# cookie-attribute had an initial dot, in order to follow RFC 2965
# (as clarified in draft errata). Needed for the returned $Domain
# value.
self.domain_initial_dot = domain_initial_dot
self.path = path
self.path_specified = path_specified
self.secure = secure
self.expires = expires
self.discard = discard
self.comment = comment
self.comment_url = comment_url
self.rfc2109 = rfc2109
self._rest = copy.copy(rest)
def has_nonstandard_attr(self, name):
return name in self._rest
def get_nonstandard_attr(self, name, default=None):
return self._rest.get(name, default)
def set_nonstandard_attr(self, name, value):
self._rest[name] = value
def is_expired(self, now=None):
if now is None: now = time.time()
if (self.expires is not None) and (self.expires <= now):
return True
return False
def __str__(self):
if self.port is None: p = ""
else: p = ":"+self.port
limit = self.domain + p + self.path
if self.value is not None:
namevalue = "%s=%s" % (self.name, self.value)
else:
namevalue = self.name
return "<Cookie %s for %s>" % (namevalue, limit)
def __repr__(self):
args = []
for name in ("version", "name", "value",
"port", "port_specified",
"domain", "domain_specified", "domain_initial_dot",
"path", "path_specified",
"secure", "expires", "discard", "comment", "comment_url",
):
attr = getattr(self, name)
args.append("%s=%s" % (name, repr(attr)))
args.append("rest=%s" % repr(self._rest))
args.append("rfc2109=%s" % repr(self.rfc2109))
return "Cookie(%s)" % ", ".join(args)
class CookiePolicy:
"""Defines which cookies get accepted from and returned to server.
May also modify cookies, though this is probably a bad idea.
The subclass DefaultCookiePolicy defines the standard rules for Netscape
and RFC 2965 cookies -- override that if you want a customised policy.
"""
def set_ok(self, cookie, request):
"""Return true if (and only if) cookie should be accepted from server.
Currently, pre-expired cookies never get this far -- the CookieJar
class deletes such cookies itself.
"""
raise NotImplementedError()
def return_ok(self, cookie, request):
"""Return true if (and only if) cookie should be returned to server."""
raise NotImplementedError()
def domain_return_ok(self, domain, request):
"""Return false if cookies should not be returned, given cookie domain.
"""
return True
def path_return_ok(self, path, request):
"""Return false if cookies should not be returned, given cookie path.
"""
return True
class DefaultCookiePolicy(CookiePolicy):
"""Implements the standard rules for accepting and returning cookies."""
DomainStrictNoDots = 1
DomainStrictNonDomain = 2
DomainRFC2965Match = 4
DomainLiberal = 0
DomainStrict = DomainStrictNoDots|DomainStrictNonDomain
def __init__(self,
blocked_domains=None, allowed_domains=None,
netscape=True, rfc2965=False,
rfc2109_as_netscape=None,
hide_cookie2=False,
strict_domain=False,
strict_rfc2965_unverifiable=True,
strict_ns_unverifiable=False,
strict_ns_domain=DomainLiberal,
strict_ns_set_initial_dollar=False,
strict_ns_set_path=False,
):
"""Constructor arguments should be passed as keyword arguments only."""
self.netscape = netscape
self.rfc2965 = rfc2965
self.rfc2109_as_netscape = rfc2109_as_netscape
self.hide_cookie2 = hide_cookie2
self.strict_domain = strict_domain
self.strict_rfc2965_unverifiable = strict_rfc2965_unverifiable
self.strict_ns_unverifiable = strict_ns_unverifiable
self.strict_ns_domain = strict_ns_domain
self.strict_ns_set_initial_dollar = strict_ns_set_initial_dollar
self.strict_ns_set_path = strict_ns_set_path
if blocked_domains is not None:
self._blocked_domains = tuple(blocked_domains)
else:
self._blocked_domains = ()
if allowed_domains is not None:
allowed_domains = tuple(allowed_domains)
self._allowed_domains = allowed_domains
def blocked_domains(self):
"""Return the sequence of blocked domains (as a tuple)."""
return self._blocked_domains
def set_blocked_domains(self, blocked_domains):
"""Set the sequence of blocked domains."""
self._blocked_domains = tuple(blocked_domains)
def is_blocked(self, domain):
for blocked_domain in self._blocked_domains:
if user_domain_match(domain, blocked_domain):
return True
return False
def allowed_domains(self):
"""Return None, or the sequence of allowed domains (as a tuple)."""
return self._allowed_domains
def set_allowed_domains(self, allowed_domains):
"""Set the sequence of allowed domains, or None."""
if allowed_domains is not None:
allowed_domains = tuple(allowed_domains)
self._allowed_domains = allowed_domains
def is_not_allowed(self, domain):
if self._allowed_domains is None:
return False
for allowed_domain in self._allowed_domains:
if user_domain_match(domain, allowed_domain):
return False
return True
def set_ok(self, cookie, request):
"""
If you override .set_ok(), be sure to call this method. If it returns
false, so should your subclass (assuming your subclass wants to be more
strict about which cookies to accept).
"""
_debug(" - checking cookie %s=%s", cookie.name, cookie.value)
assert cookie.name is not None
for n in "version", "verifiability", "name", "path", "domain", "port":
fn_name = "set_ok_"+n
fn = getattr(self, fn_name)
if not fn(cookie, request):
return False
return True
def set_ok_version(self, cookie, request):
if cookie.version is None:
# Version is always set to 0 by parse_ns_headers if it's a Netscape
# cookie, so this must be an invalid RFC 2965 cookie.
_debug(" Set-Cookie2 without version attribute (%s=%s)",
cookie.name, cookie.value)
return False
if cookie.version > 0 and not self.rfc2965:
_debug(" RFC 2965 cookies are switched off")
return False
elif cookie.version == 0 and not self.netscape:
_debug(" Netscape cookies are switched off")
return False
return True
def set_ok_verifiability(self, cookie, request):
if request.unverifiable and is_third_party(request):
if cookie.version > 0 and self.strict_rfc2965_unverifiable:
_debug(" third-party RFC 2965 cookie during "
"unverifiable transaction")
return False
elif cookie.version == 0 and self.strict_ns_unverifiable:
_debug(" third-party Netscape cookie during "
"unverifiable transaction")
return False
return True
def set_ok_name(self, cookie, request):
# Try and stop servers setting V0 cookies designed to hack other
# servers that know both V0 and V1 protocols.
if (cookie.version == 0 and self.strict_ns_set_initial_dollar and
cookie.name.startswith("$")):
_debug(" illegal name (starts with '$'): '%s'", cookie.name)
return False
return True
def set_ok_path(self, cookie, request):
if cookie.path_specified:
req_path = request_path(request)
if ((cookie.version > 0 or
(cookie.version == 0 and self.strict_ns_set_path)) and
not req_path.startswith(cookie.path)):
_debug(" path attribute %s is not a prefix of request "
"path %s", cookie.path, req_path)
return False
return True
def set_ok_domain(self, cookie, request):
if self.is_blocked(cookie.domain):
_debug(" domain %s is in user block-list", cookie.domain)
return False
if self.is_not_allowed(cookie.domain):
_debug(" domain %s is not in user allow-list", cookie.domain)
return False
if cookie.domain_specified:
req_host, erhn = eff_request_host(request)
domain = cookie.domain
if self.strict_domain and (domain.count(".") >= 2):
# XXX This should probably be compared with the Konqueror
# (kcookiejar.cpp) and Mozilla implementations, but it's a
# losing battle.
i = domain.rfind(".")
j = domain.rfind(".", 0, i)
if j == 0: # domain like .foo.bar
tld = domain[i+1:]
sld = domain[j+1:i]
if sld.lower() in ("co", "ac", "com", "edu", "org", "net",
"gov", "mil", "int", "aero", "biz", "cat", "coop",
"info", "jobs", "mobi", "museum", "name", "pro",
"travel", "eu") and len(tld) == 2:
# domain like .co.uk
_debug(" country-code second level domain %s", domain)
return False
if domain.startswith("."):
undotted_domain = domain[1:]
else:
undotted_domain = domain
embedded_dots = (undotted_domain.find(".") >= 0)
if not embedded_dots and domain != ".local":
_debug(" non-local domain %s contains no embedded dot",
domain)
return False
if cookie.version == 0:
if (not erhn.endswith(domain) and
(not erhn.startswith(".") and
not ("."+erhn).endswith(domain))):
_debug(" effective request-host %s (even with added "
"initial dot) does not end with %s",
erhn, domain)
return False
if (cookie.version > 0 or
(self.strict_ns_domain & self.DomainRFC2965Match)):
if not domain_match(erhn, domain):
_debug(" effective request-host %s does not domain-match "
"%s", erhn, domain)
return False
if (cookie.version > 0 or
(self.strict_ns_domain & self.DomainStrictNoDots)):
host_prefix = req_host[:-len(domain)]
if (host_prefix.find(".") >= 0 and
not IPV4_RE.search(req_host)):
_debug(" host prefix %s for domain %s contains a dot",
host_prefix, domain)
return False
return True
def set_ok_port(self, cookie, request):
if cookie.port_specified:
req_port = request_port(request)
if req_port is None:
req_port = "80"
else:
req_port = str(req_port)
for p in cookie.port.split(","):
try:
int(p)
except ValueError:
_debug(" bad port %s (not numeric)", p)
return False
if p == req_port:
break
else:
_debug(" request port (%s) not found in %s",
req_port, cookie.port)
return False
return True
def return_ok(self, cookie, request):
"""
If you override .return_ok(), be sure to call this method. If it
returns false, so should your subclass (assuming your subclass wants to
be more strict about which cookies to return).
"""
# Path has already been checked by .path_return_ok(), and domain
# blocking done by .domain_return_ok().
_debug(" - checking cookie %s=%s", cookie.name, cookie.value)
for n in "version", "verifiability", "secure", "expires", "port", "domain":
fn_name = "return_ok_"+n
fn = getattr(self, fn_name)
if not fn(cookie, request):
return False
return True
def return_ok_version(self, cookie, request):
if cookie.version > 0 and not self.rfc2965:
_debug(" RFC 2965 cookies are switched off")
return False
elif cookie.version == 0 and not self.netscape:
_debug(" Netscape cookies are switched off")
return False
return True
def return_ok_verifiability(self, cookie, request):
if request.unverifiable and is_third_party(request):
if cookie.version > 0 and self.strict_rfc2965_unverifiable:
_debug(" third-party RFC 2965 cookie during unverifiable "
"transaction")
return False
elif cookie.version == 0 and self.strict_ns_unverifiable:
_debug(" third-party Netscape cookie during unverifiable "
"transaction")
return False
return True
def return_ok_secure(self, cookie, request):
if cookie.secure and request.type != "https":
_debug(" secure cookie with non-secure request")
return False
return True
def return_ok_expires(self, cookie, request):
if cookie.is_expired(self._now):
_debug(" cookie expired")
return False
return True
def return_ok_port(self, cookie, request):
if cookie.port:
req_port = request_port(request)
if req_port is None:
req_port = "80"
for p in cookie.port.split(","):
if p == req_port:
break
else:
_debug(" request port %s does not match cookie port %s",
req_port, cookie.port)
return False
return True
def return_ok_domain(self, cookie, request):
req_host, erhn = eff_request_host(request)
domain = cookie.domain
# strict check of non-domain cookies: Mozilla does this, MSIE5 doesn't
if (cookie.version == 0 and
(self.strict_ns_domain & self.DomainStrictNonDomain) and
not cookie.domain_specified and domain != erhn):
_debug(" cookie with unspecified domain does not string-compare "
"equal to request domain")
return False
if cookie.version > 0 and not domain_match(erhn, domain):
_debug(" effective request-host name %s does not domain-match "
"RFC 2965 cookie domain %s", erhn, domain)
return False
if cookie.version == 0 and not ("."+erhn).endswith(domain):
_debug(" request-host %s does not match Netscape cookie domain "
"%s", req_host, domain)
return False
return True
def domain_return_ok(self, domain, request):
# Liberal check of. This is here as an optimization to avoid
# having to load lots of MSIE cookie files unless necessary.
req_host, erhn = eff_request_host(request)
if not req_host.startswith("."):
req_host = "."+req_host
if not erhn.startswith("."):
erhn = "."+erhn
if not (req_host.endswith(domain) or erhn.endswith(domain)):
#_debug(" request domain %s does not match cookie domain %s",
# req_host, domain)
return False
if self.is_blocked(domain):
_debug(" domain %s is in user block-list", domain)
return False
if self.is_not_allowed(domain):
_debug(" domain %s is not in user allow-list", domain)
return False
return True
def path_return_ok(self, path, request):
_debug("- checking cookie path=%s", path)
req_path = request_path(request)
if not req_path.startswith(path):
_debug(" %s does not path-match %s", req_path, path)
return False
return True
def vals_sorted_by_key(adict):
keys = sorted(adict.keys())
return map(adict.get, keys)
def deepvalues(mapping):
"""Iterates over nested mapping, depth-first, in sorted order by key."""
values = vals_sorted_by_key(mapping)
for obj in values:
mapping = False
try:
obj.items
except AttributeError:
pass
else:
mapping = True
for subobj in deepvalues(obj):
yield subobj
if not mapping:
yield obj
# Used as second parameter to dict.get() method, to distinguish absent
# dict key from one with a None value.
class Absent: pass
class CookieJar:
"""Collection of HTTP cookies.
You may not need to know about this class: try
urllib.request.build_opener(HTTPCookieProcessor).open(url).
"""
non_word_re = re.compile(r"\W")
quote_re = re.compile(r"([\"\\])")
strict_domain_re = re.compile(r"\.?[^.]*")
domain_re = re.compile(r"[^.]*")
dots_re = re.compile(r"^\.+")
magic_re = re.compile(r"^\#LWP-Cookies-(\d+\.\d+)", re.ASCII)
def __init__(self, policy=None):
if policy is None:
policy = DefaultCookiePolicy()
self._policy = policy
self._cookies_lock = _threading.RLock()
self._cookies = {}
def set_policy(self, policy):
self._policy = policy
def _cookies_for_domain(self, domain, request):
cookies = []
if not self._policy.domain_return_ok(domain, request):
return []
_debug("Checking %s for cookies to return", domain)
cookies_by_path = self._cookies[domain]
for path in cookies_by_path.keys():
if not self._policy.path_return_ok(path, request):
continue
cookies_by_name = cookies_by_path[path]
for cookie in cookies_by_name.values():
if not self._policy.return_ok(cookie, request):
_debug(" not returning cookie")
continue
_debug(" it's a match")
cookies.append(cookie)
return cookies
def _cookies_for_request(self, request):
"""Return a list of cookies to be returned to server."""
cookies = []
for domain in self._cookies.keys():
cookies.extend(self._cookies_for_domain(domain, request))
return cookies
def _cookie_attrs(self, cookies):
"""Return a list of cookie-attributes to be returned to server.
like ['foo="bar"; $Path="/"', ...]
The $Version attribute is also added when appropriate (currently only
once per request).
"""
# add cookies in order of most specific (ie. longest) path first
cookies.sort(key=lambda a: len(a.path), reverse=True)
version_set = False
attrs = []
for cookie in cookies:
# set version of Cookie header
# XXX
# What should it be if multiple matching Set-Cookie headers have
# different versions themselves?
# Answer: there is no answer; was supposed to be settled by
# RFC 2965 errata, but that may never appear...
version = cookie.version
if not version_set:
version_set = True
if version > 0:
attrs.append("$Version=%s" % version)
# quote cookie value if necessary
# (not for Netscape protocol, which already has any quotes
# intact, due to the poorly-specified Netscape Cookie: syntax)
if ((cookie.value is not None) and
self.non_word_re.search(cookie.value) and version > 0):
value = self.quote_re.sub(r"\\\1", cookie.value)
else:
value = cookie.value
# add cookie-attributes to be returned in Cookie header
if cookie.value is None:
attrs.append(cookie.name)
else:
attrs.append("%s=%s" % (cookie.name, value))
if version > 0:
if cookie.path_specified:
attrs.append('$Path="%s"' % cookie.path)
if cookie.domain.startswith("."):
domain = cookie.domain
if (not cookie.domain_initial_dot and
domain.startswith(".")):
domain = domain[1:]
attrs.append('$Domain="%s"' % domain)
if cookie.port is not None:
p = "$Port"
if cookie.port_specified:
p = p + ('="%s"' % cookie.port)
attrs.append(p)
return attrs
def add_cookie_header(self, request):
"""Add correct Cookie: header to request (urllib.request.Request object).
The Cookie2 header is also added unless policy.hide_cookie2 is true.
"""
_debug("add_cookie_header")
self._cookies_lock.acquire()
try:
self._policy._now = self._now = int(time.time())
cookies = self._cookies_for_request(request)
attrs = self._cookie_attrs(cookies)
if attrs:
if not request.has_header("Cookie"):
request.add_unredirected_header(
"Cookie", "; ".join(attrs))
# if necessary, advertise that we know RFC 2965
if (self._policy.rfc2965 and not self._policy.hide_cookie2 and
not request.has_header("Cookie2")):
for cookie in cookies:
if cookie.version != 1:
request.add_unredirected_header("Cookie2", '$Version="1"')
break
finally:
self._cookies_lock.release()
self.clear_expired_cookies()
def _normalized_cookie_tuples(self, attrs_set):
"""Return list of tuples containing normalised cookie information.
attrs_set is the list of lists of key,value pairs extracted from
the Set-Cookie or Set-Cookie2 headers.
Tuples are name, value, standard, rest, where name and value are the
cookie name and value, standard is a dictionary containing the standard
cookie-attributes (discard, secure, version, expires or max-age,
domain, path and port) and rest is a dictionary containing the rest of
the cookie-attributes.
"""
cookie_tuples = []
boolean_attrs = "discard", "secure"
value_attrs = ("version",
"expires", "max-age",
"domain", "path", "port",
"comment", "commenturl")
for cookie_attrs in attrs_set:
name, value = cookie_attrs[0]
# Build dictionary of standard cookie-attributes (standard) and
# dictionary of other cookie-attributes (rest).
# Note: expiry time is normalised to seconds since epoch. V0
# cookies should have the Expires cookie-attribute, and V1 cookies
# should have Max-Age, but since V1 includes RFC 2109 cookies (and
# since V0 cookies may be a mish-mash of Netscape and RFC 2109), we
# accept either (but prefer Max-Age).
max_age_set = False
bad_cookie = False
standard = {}
rest = {}
for k, v in cookie_attrs[1:]:
lc = k.lower()
# don't lose case distinction for unknown fields
if lc in value_attrs or lc in boolean_attrs:
k = lc
if k in boolean_attrs and v is None:
# boolean cookie-attribute is present, but has no value
# (like "discard", rather than "port=80")
v = True
if k in standard:
# only first value is significant
continue
if k == "domain":
if v is None:
_debug(" missing value for domain attribute")
bad_cookie = True
break
# RFC 2965 section 3.3.3
v = v.lower()
if k == "expires":
if max_age_set:
# Prefer max-age to expires (like Mozilla)
continue
if v is None:
_debug(" missing or invalid value for expires "
"attribute: treating as session cookie")
continue
if k == "max-age":
max_age_set = True
try:
v = int(v)
except ValueError:
_debug(" missing or invalid (non-numeric) value for "
"max-age attribute")
bad_cookie = True
break
# convert RFC 2965 Max-Age to seconds since epoch
# XXX Strictly you're supposed to follow RFC 2616
# age-calculation rules. Remember that zero Max-Age is a
# is a request to discard (old and new) cookie, though.
k = "expires"
v = self._now + v
if (k in value_attrs) or (k in boolean_attrs):
if (v is None and
k not in ("port", "comment", "commenturl")):
_debug(" missing value for %s attribute" % k)
bad_cookie = True
break
standard[k] = v
else:
rest[k] = v
if bad_cookie:
continue
cookie_tuples.append((name, value, standard, rest))
return cookie_tuples
def _cookie_from_cookie_tuple(self, tup, request):
# standard is dict of standard cookie-attributes, rest is dict of the
# rest of them
name, value, standard, rest = tup
domain = standard.get("domain", Absent)
path = standard.get("path", Absent)
port = standard.get("port", Absent)
expires = standard.get("expires", Absent)
# set the easy defaults
version = standard.get("version", None)
if version is not None:
try:
version = int(version)
except ValueError:
return None # invalid version, ignore cookie
secure = standard.get("secure", False)
# (discard is also set if expires is Absent)
discard = standard.get("discard", False)
comment = standard.get("comment", None)
comment_url = standard.get("commenturl", None)
# set default path
if path is not Absent and path != "":
path_specified = True
path = escape_path(path)
else:
path_specified = False
path = request_path(request)
i = path.rfind("/")
if i != -1:
if version == 0:
# Netscape spec parts company from reality here
path = path[:i]
else:
path = path[:i+1]
if len(path) == 0: path = "/"
# set default domain
domain_specified = domain is not Absent
# but first we have to remember whether it starts with a dot
domain_initial_dot = False
if domain_specified:
domain_initial_dot = bool(domain.startswith("."))
if domain is Absent:
req_host, erhn = eff_request_host(request)
domain = erhn
elif not domain.startswith("."):
domain = "."+domain
# set default port
port_specified = False
if port is not Absent:
if port is None:
# Port attr present, but has no value: default to request port.
# Cookie should then only be sent back on that port.
port = request_port(request)
else:
port_specified = True
port = re.sub(r"\s+", "", port)
else:
# No port attr present. Cookie can be sent back on any port.
port = None
# set default expires and discard
if expires is Absent:
expires = None
discard = True
elif expires <= self._now:
# Expiry date in past is request to delete cookie. This can't be
# in DefaultCookiePolicy, because can't delete cookies there.
try:
self.clear(domain, path, name)
except KeyError:
pass
_debug("Expiring cookie, domain='%s', path='%s', name='%s'",
domain, path, name)
return None
return Cookie(version,
name, value,
port, port_specified,
domain, domain_specified, domain_initial_dot,
path, path_specified,
secure,
expires,
discard,
comment,
comment_url,
rest)
def _cookies_from_attrs_set(self, attrs_set, request):
cookie_tuples = self._normalized_cookie_tuples(attrs_set)
cookies = []
for tup in cookie_tuples:
cookie = self._cookie_from_cookie_tuple(tup, request)
if cookie: cookies.append(cookie)
return cookies
def _process_rfc2109_cookies(self, cookies):
rfc2109_as_ns = getattr(self._policy, 'rfc2109_as_netscape', None)
if rfc2109_as_ns is None:
rfc2109_as_ns = not self._policy.rfc2965
for cookie in cookies:
if cookie.version == 1:
cookie.rfc2109 = True
if rfc2109_as_ns:
# treat 2109 cookies as Netscape cookies rather than
# as RFC2965 cookies
cookie.version = 0
def make_cookies(self, response, request):
"""Return sequence of Cookie objects extracted from response object."""
# get cookie-attributes for RFC 2965 and Netscape protocols
headers = response.info()
rfc2965_hdrs = headers.get_all("Set-Cookie2", [])
ns_hdrs = headers.get_all("Set-Cookie", [])
rfc2965 = self._policy.rfc2965
netscape = self._policy.netscape
if ((not rfc2965_hdrs and not ns_hdrs) or
(not ns_hdrs and not rfc2965) or
(not rfc2965_hdrs and not netscape) or
(not netscape and not rfc2965)):
return [] # no relevant cookie headers: quick exit
try:
cookies = self._cookies_from_attrs_set(
split_header_words(rfc2965_hdrs), request)
except Exception:
_warn_unhandled_exception()
cookies = []
if ns_hdrs and netscape:
try:
# RFC 2109 and Netscape cookies
ns_cookies = self._cookies_from_attrs_set(
parse_ns_headers(ns_hdrs), request)
except Exception:
_warn_unhandled_exception()
ns_cookies = []
self._process_rfc2109_cookies(ns_cookies)
# Look for Netscape cookies (from Set-Cookie headers) that match
# corresponding RFC 2965 cookies (from Set-Cookie2 headers).
# For each match, keep the RFC 2965 cookie and ignore the Netscape
# cookie (RFC 2965 section 9.1). Actually, RFC 2109 cookies are
# bundled in with the Netscape cookies for this purpose, which is
# reasonable behaviour.
if rfc2965:
lookup = {}
for cookie in cookies:
lookup[(cookie.domain, cookie.path, cookie.name)] = None
def no_matching_rfc2965(ns_cookie, lookup=lookup):
key = ns_cookie.domain, ns_cookie.path, ns_cookie.name
return key not in lookup
ns_cookies = filter(no_matching_rfc2965, ns_cookies)
if ns_cookies:
cookies.extend(ns_cookies)
return cookies
def set_cookie_if_ok(self, cookie, request):
"""Set a cookie if policy says it's OK to do so."""
self._cookies_lock.acquire()
try:
self._policy._now = self._now = int(time.time())
if self._policy.set_ok(cookie, request):
self.set_cookie(cookie)
finally:
self._cookies_lock.release()
def set_cookie(self, cookie):
"""Set a cookie, without checking whether or not it should be set."""
c = self._cookies
self._cookies_lock.acquire()
try:
if cookie.domain not in c: c[cookie.domain] = {}
c2 = c[cookie.domain]
if cookie.path not in c2: c2[cookie.path] = {}
c3 = c2[cookie.path]
c3[cookie.name] = cookie
finally:
self._cookies_lock.release()
def extract_cookies(self, response, request):
"""Extract cookies from response, where allowable given the request."""
_debug("extract_cookies: %s", response.info())
self._cookies_lock.acquire()
try:
self._policy._now = self._now = int(time.time())
for cookie in self.make_cookies(response, request):
if self._policy.set_ok(cookie, request):
_debug(" setting cookie: %s", cookie)
self.set_cookie(cookie)
finally:
self._cookies_lock.release()
def clear(self, domain=None, path=None, name=None):
"""Clear some cookies.
Invoking this method without arguments will clear all cookies. If
given a single argument, only cookies belonging to that domain will be
removed. If given two arguments, cookies belonging to the specified
path within that domain are removed. If given three arguments, then
the cookie with the specified name, path and domain is removed.
Raises KeyError if no matching cookie exists.
"""
if name is not None:
if (domain is None) or (path is None):
raise ValueError(
"domain and path must be given to remove a cookie by name")
del self._cookies[domain][path][name]
elif path is not None:
if domain is None:
raise ValueError(
"domain must be given to remove cookies by path")
del self._cookies[domain][path]
elif domain is not None:
del self._cookies[domain]
else:
self._cookies = {}
def clear_session_cookies(self):
"""Discard all session cookies.
Note that the .save() method won't save session cookies anyway, unless
you ask otherwise by passing a true ignore_discard argument.
"""
self._cookies_lock.acquire()
try:
for cookie in self:
if cookie.discard:
self.clear(cookie.domain, cookie.path, cookie.name)
finally:
self._cookies_lock.release()
def clear_expired_cookies(self):
"""Discard all expired cookies.
You probably don't need to call this method: expired cookies are never
sent back to the server (provided you're using DefaultCookiePolicy),
this method is called by CookieJar itself every so often, and the
.save() method won't save expired cookies anyway (unless you ask
otherwise by passing a true ignore_expires argument).
"""
self._cookies_lock.acquire()
try:
now = time.time()
for cookie in self:
if cookie.is_expired(now):
self.clear(cookie.domain, cookie.path, cookie.name)
finally:
self._cookies_lock.release()
def __iter__(self):
return deepvalues(self._cookies)
def __len__(self):
"""Return number of contained cookies."""
i = 0
for cookie in self: i = i + 1
return i
def __repr__(self):
r = []
for cookie in self: r.append(repr(cookie))
return "<%s[%s]>" % (self.__class__, ", ".join(r))
def __str__(self):
r = []
for cookie in self: r.append(str(cookie))
return "<%s[%s]>" % (self.__class__, ", ".join(r))
# derives from IOError for backwards-compatibility with Python 2.4.0
class LoadError(IOError): pass
class FileCookieJar(CookieJar):
"""CookieJar that can be loaded from and saved to a file."""
def __init__(self, filename=None, delayload=False, policy=None):
"""
Cookies are NOT loaded from the named file until either the .load() or
.revert() method is called.
"""
CookieJar.__init__(self, policy)
if filename is not None:
try:
filename+""
except:
raise ValueError("filename must be string-like")
self.filename = filename
self.delayload = bool(delayload)
def save(self, filename=None, ignore_discard=False, ignore_expires=False):
"""Save cookies to a file."""
raise NotImplementedError()
def load(self, filename=None, ignore_discard=False, ignore_expires=False):
"""Load cookies from a file."""
if filename is None:
if self.filename is not None: filename = self.filename
else: raise ValueError(MISSING_FILENAME_TEXT)
f = open(filename)
try:
self._really_load(f, filename, ignore_discard, ignore_expires)
finally:
f.close()
def revert(self, filename=None,
ignore_discard=False, ignore_expires=False):
"""Clear all cookies and reload cookies from a saved file.
Raises LoadError (or IOError) if reversion is not successful; the
object's state will not be altered if this happens.
"""
if filename is None:
if self.filename is not None: filename = self.filename
else: raise ValueError(MISSING_FILENAME_TEXT)
self._cookies_lock.acquire()
try:
old_state = copy.deepcopy(self._cookies)
self._cookies = {}
try:
self.load(filename, ignore_discard, ignore_expires)
except (LoadError, IOError):
self._cookies = old_state
raise
finally:
self._cookies_lock.release()
def lwp_cookie_str(cookie):
"""Return string representation of Cookie in an the LWP cookie file format.
Actually, the format is extended a bit -- see module docstring.
"""
h = [(cookie.name, cookie.value),
("path", cookie.path),
("domain", cookie.domain)]
if cookie.port is not None: h.append(("port", cookie.port))
if cookie.path_specified: h.append(("path_spec", None))
if cookie.port_specified: h.append(("port_spec", None))
if cookie.domain_initial_dot: h.append(("domain_dot", None))
if cookie.secure: h.append(("secure", None))
if cookie.expires: h.append(("expires",
time2isoz(float(cookie.expires))))
if cookie.discard: h.append(("discard", None))
if cookie.comment: h.append(("comment", cookie.comment))
if cookie.comment_url: h.append(("commenturl", cookie.comment_url))
keys = sorted(cookie._rest.keys())
for k in keys:
h.append((k, str(cookie._rest[k])))
h.append(("version", str(cookie.version)))
return join_header_words([h])
class LWPCookieJar(FileCookieJar):
"""
The LWPCookieJar saves a sequence of "Set-Cookie3" lines.
"Set-Cookie3" is the format used by the libwww-perl libary, not known
to be compatible with any browser, but which is easy to read and
doesn't lose information about RFC 2965 cookies.
Additional methods
as_lwp_str(ignore_discard=True, ignore_expired=True)
"""
def as_lwp_str(self, ignore_discard=True, ignore_expires=True):
"""Return cookies as a string of "\\n"-separated "Set-Cookie3" headers.
ignore_discard and ignore_expires: see docstring for FileCookieJar.save
"""
now = time.time()
r = []
for cookie in self:
if not ignore_discard and cookie.discard:
continue
if not ignore_expires and cookie.is_expired(now):
continue
r.append("Set-Cookie3: %s" % lwp_cookie_str(cookie))
return "\n".join(r+[""])
def save(self, filename=None, ignore_discard=False, ignore_expires=False):
if filename is None:
if self.filename is not None: filename = self.filename
else: raise ValueError(MISSING_FILENAME_TEXT)
f = open(filename, "w")
try:
# There really isn't an LWP Cookies 2.0 format, but this indicates
# that there is extra information in here (domain_dot and
# port_spec) while still being compatible with libwww-perl, I hope.
f.write("#LWP-Cookies-2.0\n")
f.write(self.as_lwp_str(ignore_discard, ignore_expires))
finally:
f.close()
def _really_load(self, f, filename, ignore_discard, ignore_expires):
magic = f.readline()
if not self.magic_re.search(magic):
msg = ("%r does not look like a Set-Cookie3 (LWP) format "
"file" % filename)
raise LoadError(msg)
now = time.time()
header = "Set-Cookie3:"
boolean_attrs = ("port_spec", "path_spec", "domain_dot",
"secure", "discard")
value_attrs = ("version",
"port", "path", "domain",
"expires",
"comment", "commenturl")
try:
while 1:
line = f.readline()
if line == "": break
if not line.startswith(header):
continue
line = line[len(header):].strip()
for data in split_header_words([line]):
name, value = data[0]
standard = {}
rest = {}
for k in boolean_attrs:
standard[k] = False
for k, v in data[1:]:
if k is not None:
lc = k.lower()
else:
lc = None
# don't lose case distinction for unknown fields
if (lc in value_attrs) or (lc in boolean_attrs):
k = lc
if k in boolean_attrs:
if v is None: v = True
standard[k] = v
elif k in value_attrs:
standard[k] = v
else:
rest[k] = v
h = standard.get
expires = h("expires")
discard = h("discard")
if expires is not None:
expires = iso2time(expires)
if expires is None:
discard = True
domain = h("domain")
domain_specified = domain.startswith(".")
c = Cookie(h("version"), name, value,
h("port"), h("port_spec"),
domain, domain_specified, h("domain_dot"),
h("path"), h("path_spec"),
h("secure"),
expires,
discard,
h("comment"),
h("commenturl"),
rest)
if not ignore_discard and c.discard:
continue
if not ignore_expires and c.is_expired(now):
continue
self.set_cookie(c)
except IOError:
raise
except Exception:
_warn_unhandled_exception()
raise LoadError("invalid Set-Cookie3 format file %r: %r" %
(filename, line))
class MozillaCookieJar(FileCookieJar):
"""
WARNING: you may want to backup your browser's cookies file if you use
this class to save cookies. I *think* it works, but there have been
bugs in the past!
This class differs from CookieJar only in the format it uses to save and
load cookies to and from a file. This class uses the Mozilla/Netscape
`cookies.txt' format. lynx uses this file format, too.
Don't expect cookies saved while the browser is running to be noticed by
the browser (in fact, Mozilla on unix will overwrite your saved cookies if
you change them on disk while it's running; on Windows, you probably can't
save at all while the browser is running).
Note that the Mozilla/Netscape format will downgrade RFC2965 cookies to
Netscape cookies on saving.
In particular, the cookie version and port number information is lost,
together with information about whether or not Path, Port and Discard were
specified by the Set-Cookie2 (or Set-Cookie) header, and whether or not the
domain as set in the HTTP header started with a dot (yes, I'm aware some
domains in Netscape files start with a dot and some don't -- trust me, you
really don't want to know any more about this).
Note that though Mozilla and Netscape use the same format, they use
slightly different headers. The class saves cookies using the Netscape
header by default (Mozilla can cope with that).
"""
magic_re = re.compile("#( Netscape)? HTTP Cookie File")
header = """\
# Netscape HTTP Cookie File
# http://curl.haxx.se/rfc/cookie_spec.html
# This is a generated file! Do not edit.
"""
def _really_load(self, f, filename, ignore_discard, ignore_expires):
now = time.time()
magic = f.readline()
if not self.magic_re.search(magic):
f.close()
raise LoadError(
"%r does not look like a Netscape format cookies file" %
filename)
try:
while 1:
line = f.readline()
if line == "": break
# last field may be absent, so keep any trailing tab
if line.endswith("\n"): line = line[:-1]
# skip comments and blank lines XXX what is $ for?
if (line.strip().startswith(("#", "$")) or
line.strip() == ""):
continue
domain, domain_specified, path, secure, expires, name, value = \
line.split("\t")
secure = (secure == "TRUE")
domain_specified = (domain_specified == "TRUE")
if name == "":
# cookies.txt regards 'Set-Cookie: foo' as a cookie
# with no name, whereas http.cookiejar regards it as a
# cookie with no value.
name = value
value = None
initial_dot = domain.startswith(".")
assert domain_specified == initial_dot
discard = False
if expires == "":
expires = None
discard = True
# assume path_specified is false
c = Cookie(0, name, value,
None, False,
domain, domain_specified, initial_dot,
path, False,
secure,
expires,
discard,
None,
None,
{})
if not ignore_discard and c.discard:
continue
if not ignore_expires and c.is_expired(now):
continue
self.set_cookie(c)
except IOError:
raise
except Exception:
_warn_unhandled_exception()
raise LoadError("invalid Netscape format cookies file %r: %r" %
(filename, line))
def save(self, filename=None, ignore_discard=False, ignore_expires=False):
if filename is None:
if self.filename is not None: filename = self.filename
else: raise ValueError(MISSING_FILENAME_TEXT)
f = open(filename, "w")
try:
f.write(self.header)
now = time.time()
for cookie in self:
if not ignore_discard and cookie.discard:
continue
if not ignore_expires and cookie.is_expired(now):
continue
if cookie.secure: secure = "TRUE"
else: secure = "FALSE"
if cookie.domain.startswith("."): initial_dot = "TRUE"
else: initial_dot = "FALSE"
if cookie.expires is not None:
expires = str(cookie.expires)
else:
expires = ""
if cookie.value is None:
# cookies.txt regards 'Set-Cookie: foo' as a cookie
# with no name, whereas http.cookiejar regards it as a
# cookie with no value.
name = ""
value = cookie.name
else:
name = cookie.name
value = cookie.value
f.write(
"\t".join([cookie.domain, initial_dot, cookie.path,
secure, expires, name, value])+
"\n")
finally:
f.close()
| {'content_hash': '559998a8add628988658911617f859c8', 'timestamp': '', 'source': 'github', 'line_count': 2091, 'max_line_length': 83, 'avg_line_length': 36.324725011956005, 'alnum_prop': 0.5385425580936081, 'repo_name': 'timm/timmnix', 'id': '9fcd4c6f462e47f869f7cf67d496da38d7faf527', 'size': '75955', 'binary': False, 'copies': '3', 'ref': 'refs/heads/master', 'path': 'pypy3-v5.5.0-linux64/lib-python/3/http/cookiejar.py', 'mode': '33261', 'license': 'mit', 'language': [{'name': 'Assembly', 'bytes': '1641'}, {'name': 'Batchfile', 'bytes': '1234'}, {'name': 'C', 'bytes': '436685'}, {'name': 'CSS', 'bytes': '96'}, {'name': 'Common Lisp', 'bytes': '4'}, {'name': 'Emacs Lisp', 'bytes': '290698'}, {'name': 'HTML', 'bytes': '111577'}, {'name': 'Makefile', 'bytes': '1681'}, {'name': 'PLSQL', 'bytes': '22886'}, {'name': 'PowerShell', 'bytes': '1540'}, {'name': 'Prolog', 'bytes': '14301'}, {'name': 'Python', 'bytes': '21267592'}, {'name': 'Roff', 'bytes': '21080'}, {'name': 'Shell', 'bytes': '27687'}, {'name': 'TeX', 'bytes': '3052861'}, {'name': 'VBScript', 'bytes': '481'}]} |
package org.mule.tooling.esb.lang.mel.highlighter;
import com.intellij.openapi.fileTypes.SyntaxHighlighter;
import com.intellij.openapi.fileTypes.SyntaxHighlighterFactory;
import com.intellij.openapi.project.Project;
import com.intellij.openapi.vfs.VirtualFile;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
public class MelSyntaxHighlighterFactory extends SyntaxHighlighterFactory {
@NotNull
@Override
public SyntaxHighlighter getSyntaxHighlighter(@Nullable Project project, @Nullable VirtualFile virtualFile) {
return MelSyntaxHighlighter.getInstance();
}
}
| {'content_hash': 'bb842a07b7fb793876f129aaa7c8d4a8', 'timestamp': '', 'source': 'github', 'line_count': 17, 'max_line_length': 113, 'avg_line_length': 36.8235294117647, 'alnum_prop': 0.8210862619808307, 'repo_name': 'machaval/mule-intellij-plugins', 'id': '9799708e729ba0ce58ab3830b4aa7889ea64ca45', 'size': '626', 'binary': False, 'copies': '2', 'ref': 'refs/heads/master', 'path': 'mule-esb-plugin/src/main/java/org/mule/tooling/esb/lang/mel/highlighter/MelSyntaxHighlighterFactory.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Java', 'bytes': '660750'}, {'name': 'Lex', 'bytes': '10081'}]} |
// Copyright (c) 2019-2020 The Bitcoin Core developers
// Distributed under the MIT software license, see the accompanying
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
#include <amount.h>
#include <arith_uint256.h>
#include <compressor.h>
#include <consensus/merkle.h>
#include <core_io.h>
#include <crypto/common.h>
#include <crypto/siphash.h>
#include <key_io.h>
#include <memusage.h>
#include <netbase.h>
#include <policy/settings.h>
#include <pow.h>
#include <protocol.h>
#include <pubkey.h>
#include <rpc/util.h>
#include <script/signingprovider.h>
#include <script/standard.h>
#include <serialize.h>
#include <streams.h>
#include <test/fuzz/FuzzedDataProvider.h>
#include <test/fuzz/fuzz.h>
#include <test/fuzz/util.h>
#include <uint256.h>
#include <util/check.h>
#include <util/moneystr.h>
#include <util/strencodings.h>
#include <util/string.h>
#include <util/system.h>
#include <util/time.h>
#include <version.h>
#include <cassert>
#include <chrono>
#include <ctime>
#include <limits>
#include <set>
#include <vector>
void initialize()
{
SelectParams(CBaseChainParams::REGTEST);
}
void test_one_input(const std::vector<uint8_t>& buffer)
{
if (buffer.size() < sizeof(uint256) + sizeof(uint160)) {
return;
}
FuzzedDataProvider fuzzed_data_provider(buffer.data(), buffer.size());
const uint256 u256(fuzzed_data_provider.ConsumeBytes<unsigned char>(sizeof(uint256)));
const uint160 u160(fuzzed_data_provider.ConsumeBytes<unsigned char>(sizeof(uint160)));
const uint64_t u64 = fuzzed_data_provider.ConsumeIntegral<uint64_t>();
const int64_t i64 = fuzzed_data_provider.ConsumeIntegral<int64_t>();
const uint32_t u32 = fuzzed_data_provider.ConsumeIntegral<uint32_t>();
const int32_t i32 = fuzzed_data_provider.ConsumeIntegral<int32_t>();
const uint16_t u16 = fuzzed_data_provider.ConsumeIntegral<uint16_t>();
const int16_t i16 = fuzzed_data_provider.ConsumeIntegral<int16_t>();
const uint8_t u8 = fuzzed_data_provider.ConsumeIntegral<uint8_t>();
const int8_t i8 = fuzzed_data_provider.ConsumeIntegral<int8_t>();
// We cannot assume a specific value of std::is_signed<char>::value:
// ConsumeIntegral<char>() instead of casting from {u,}int8_t.
const char ch = fuzzed_data_provider.ConsumeIntegral<char>();
const bool b = fuzzed_data_provider.ConsumeBool();
const Consensus::Params& consensus_params = Params().GetConsensus();
(void)CheckProofOfWork(u256, u32, consensus_params);
if (u64 <= MAX_MONEY) {
const uint64_t compressed_money_amount = CompressAmount(u64);
assert(u64 == DecompressAmount(compressed_money_amount));
static const uint64_t compressed_money_amount_max = CompressAmount(MAX_MONEY - 1);
assert(compressed_money_amount <= compressed_money_amount_max);
} else {
(void)CompressAmount(u64);
}
static const uint256 u256_min(uint256S("0000000000000000000000000000000000000000000000000000000000000000"));
static const uint256 u256_max(uint256S("ffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffff"));
const std::vector<uint256> v256{u256, u256_min, u256_max};
(void)ComputeMerkleRoot(v256);
(void)CountBits(u64);
(void)DecompressAmount(u64);
(void)FormatISO8601Date(i64);
(void)FormatISO8601DateTime(i64);
// FormatMoney(i) not defined when i == std::numeric_limits<int64_t>::min()
if (i64 != std::numeric_limits<int64_t>::min()) {
int64_t parsed_money;
if (ParseMoney(FormatMoney(i64), parsed_money)) {
assert(parsed_money == i64);
}
}
(void)GetSizeOfCompactSize(u64);
(void)GetSpecialScriptSize(u32);
if (!MultiplicationOverflow(i64, static_cast<int64_t>(::nBytesPerSigOp)) && !AdditionOverflow(i64 * ::nBytesPerSigOp, static_cast<int64_t>(4))) {
(void)GetVirtualTransactionSize(i64, i64);
}
if (!MultiplicationOverflow(i64, static_cast<int64_t>(u32)) && !AdditionOverflow(i64, static_cast<int64_t>(4)) && !AdditionOverflow(i64 * u32, static_cast<int64_t>(4))) {
(void)GetVirtualTransactionSize(i64, i64, u32);
}
(void)HexDigit(ch);
(void)MoneyRange(i64);
(void)ToString(i64);
(void)IsDigit(ch);
(void)IsSpace(ch);
(void)IsSwitchChar(ch);
(void)memusage::DynamicUsage(ch);
(void)memusage::DynamicUsage(i16);
(void)memusage::DynamicUsage(i32);
(void)memusage::DynamicUsage(i64);
(void)memusage::DynamicUsage(i8);
(void)memusage::DynamicUsage(u16);
(void)memusage::DynamicUsage(u32);
(void)memusage::DynamicUsage(u64);
(void)memusage::DynamicUsage(u8);
const unsigned char uch = static_cast<unsigned char>(u8);
(void)memusage::DynamicUsage(uch);
{
const std::set<int64_t> i64s{i64, static_cast<int64_t>(u64)};
const size_t dynamic_usage = memusage::DynamicUsage(i64s);
const size_t incremental_dynamic_usage = memusage::IncrementalDynamicUsage(i64s);
assert(dynamic_usage == incremental_dynamic_usage * i64s.size());
}
(void)MillisToTimeval(i64);
const double d = ser_uint64_to_double(u64);
assert(ser_double_to_uint64(d) == u64);
const float f = ser_uint32_to_float(u32);
assert(ser_float_to_uint32(f) == u32);
(void)SighashToStr(uch);
(void)SipHashUint256(u64, u64, u256);
(void)SipHashUint256Extra(u64, u64, u256, u32);
(void)ToLower(ch);
(void)ToUpper(ch);
// ValueFromAmount(i) not defined when i == std::numeric_limits<int64_t>::min()
if (i64 != std::numeric_limits<int64_t>::min()) {
int64_t parsed_money;
if (ParseMoney(ValueFromAmount(i64).getValStr(), parsed_money)) {
assert(parsed_money == i64);
}
}
if (i32 >= 0 && i32 <= 16) {
assert(i32 == CScript::DecodeOP_N(CScript::EncodeOP_N(i32)));
}
const std::chrono::seconds seconds{i64};
assert(count_seconds(seconds) == i64);
const CScriptNum script_num{i64};
(void)script_num.getint();
(void)script_num.getvch();
const arith_uint256 au256 = UintToArith256(u256);
assert(ArithToUint256(au256) == u256);
assert(uint256S(au256.GetHex()) == u256);
(void)au256.bits();
(void)au256.GetCompact(/* fNegative= */ false);
(void)au256.GetCompact(/* fNegative= */ true);
(void)au256.getdouble();
(void)au256.GetHex();
(void)au256.GetLow64();
(void)au256.size();
(void)au256.ToString();
const CKeyID key_id{u160};
const CScriptID script_id{u160};
// CTxDestination = CNoDestination ∪ PKHash ∪ ScriptHash ∪ WitnessV0ScriptHash ∪ WitnessV0KeyHash ∪ WitnessUnknown
const PKHash pk_hash{u160};
const ScriptHash script_hash{u160};
const WitnessV0KeyHash witness_v0_key_hash{u160};
const WitnessV0ScriptHash witness_v0_script_hash{u256};
const std::vector<CTxDestination> destinations{pk_hash, script_hash, witness_v0_key_hash, witness_v0_script_hash};
const SigningProvider store;
for (const CTxDestination& destination : destinations) {
(void)DescribeAddress(destination);
(void)EncodeDestination(destination);
(void)GetKeyForDestination(store, destination);
(void)GetScriptForDestination(destination);
(void)IsValidDestination(destination);
}
{
CDataStream stream(SER_NETWORK, INIT_PROTO_VERSION);
uint256 deserialized_u256;
stream << u256;
stream >> deserialized_u256;
assert(u256 == deserialized_u256 && stream.empty());
uint160 deserialized_u160;
stream << u160;
stream >> deserialized_u160;
assert(u160 == deserialized_u160 && stream.empty());
uint64_t deserialized_u64;
stream << u64;
stream >> deserialized_u64;
assert(u64 == deserialized_u64 && stream.empty());
int64_t deserialized_i64;
stream << i64;
stream >> deserialized_i64;
assert(i64 == deserialized_i64 && stream.empty());
uint32_t deserialized_u32;
stream << u32;
stream >> deserialized_u32;
assert(u32 == deserialized_u32 && stream.empty());
int32_t deserialized_i32;
stream << i32;
stream >> deserialized_i32;
assert(i32 == deserialized_i32 && stream.empty());
uint16_t deserialized_u16;
stream << u16;
stream >> deserialized_u16;
assert(u16 == deserialized_u16 && stream.empty());
int16_t deserialized_i16;
stream << i16;
stream >> deserialized_i16;
assert(i16 == deserialized_i16 && stream.empty());
uint8_t deserialized_u8;
stream << u8;
stream >> deserialized_u8;
assert(u8 == deserialized_u8 && stream.empty());
int8_t deserialized_i8;
stream << i8;
stream >> deserialized_i8;
assert(i8 == deserialized_i8 && stream.empty());
char deserialized_ch;
stream << ch;
stream >> deserialized_ch;
assert(ch == deserialized_ch && stream.empty());
bool deserialized_b;
stream << b;
stream >> deserialized_b;
assert(b == deserialized_b && stream.empty());
}
{
const ServiceFlags service_flags = (ServiceFlags)u64;
(void)HasAllDesirableServiceFlags(service_flags);
(void)MayHaveUsefulAddressDB(service_flags);
}
{
CDataStream stream(SER_NETWORK, INIT_PROTO_VERSION);
ser_writedata64(stream, u64);
const uint64_t deserialized_u64 = ser_readdata64(stream);
assert(u64 == deserialized_u64 && stream.empty());
ser_writedata32(stream, u32);
const uint32_t deserialized_u32 = ser_readdata32(stream);
assert(u32 == deserialized_u32 && stream.empty());
ser_writedata32be(stream, u32);
const uint32_t deserialized_u32be = ser_readdata32be(stream);
assert(u32 == deserialized_u32be && stream.empty());
ser_writedata16(stream, u16);
const uint16_t deserialized_u16 = ser_readdata16(stream);
assert(u16 == deserialized_u16 && stream.empty());
ser_writedata16be(stream, u16);
const uint16_t deserialized_u16be = ser_readdata16be(stream);
assert(u16 == deserialized_u16be && stream.empty());
ser_writedata8(stream, u8);
const uint8_t deserialized_u8 = ser_readdata8(stream);
assert(u8 == deserialized_u8 && stream.empty());
}
{
CDataStream stream(SER_NETWORK, INIT_PROTO_VERSION);
WriteCompactSize(stream, u64);
try {
const uint64_t deserialized_u64 = ReadCompactSize(stream);
assert(u64 == deserialized_u64 && stream.empty());
} catch (const std::ios_base::failure&) {
}
}
try {
CHECK_NONFATAL(b);
} catch (const NonFatalCheckError&) {
}
}
| {'content_hash': '8e4988e84d5f284afd07e22d730db100', 'timestamp': '', 'source': 'github', 'line_count': 295, 'max_line_length': 174, 'avg_line_length': 36.68813559322034, 'alnum_prop': 0.6548092026240414, 'repo_name': 'rnicoll/dogecoin', 'id': '35d6804d4f24e5048d6f1eafd3a7f0ee90a14429', 'size': '10833', 'binary': False, 'copies': '5', 'ref': 'refs/heads/main', 'path': 'src/test/fuzz/integer.cpp', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Assembly', 'bytes': '28173'}, {'name': 'C', 'bytes': '1064604'}, {'name': 'C++', 'bytes': '8101614'}, {'name': 'CMake', 'bytes': '28560'}, {'name': 'HTML', 'bytes': '21833'}, {'name': 'M4', 'bytes': '215256'}, {'name': 'Makefile', 'bytes': '117017'}, {'name': 'Objective-C++', 'bytes': '5497'}, {'name': 'Python', 'bytes': '2237402'}, {'name': 'QMake', 'bytes': '798'}, {'name': 'Sage', 'bytes': '35184'}, {'name': 'Scheme', 'bytes': '7554'}, {'name': 'Shell', 'bytes': '153769'}]} |
/** * Gets the locale for translations. * Alias for getLocale(), for BC
purpose. * * @return string $locale Locale to use for the translation,
e.g. 'fr_FR' */ public function get
<?php echo $alias ?>
() { return $this->getLocale(); }
| {'content_hash': '0ff97a7b3b45465e1402f72daf6187e0', 'timestamp': '', 'source': 'github', 'line_count': 5, 'max_line_length': 72, 'avg_line_length': 47.2, 'alnum_prop': 0.6652542372881356, 'repo_name': 'rodolfobais/proylectura', 'id': '102a40261b22a0ccf2bce21339726bfc09cda1f3', 'size': '236', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'data/vendors/propel/generator/lib/behavior/i18n/templates/objectGetLocaleAlias.php', 'mode': '33261', 'license': 'mit', 'language': [{'name': 'Batchfile', 'bytes': '310'}, {'name': 'C', 'bytes': '481532'}, {'name': 'C++', 'bytes': '6264'}, {'name': 'CSS', 'bytes': '661857'}, {'name': 'Groff', 'bytes': '60910'}, {'name': 'HTML', 'bytes': '3283009'}, {'name': 'JavaScript', 'bytes': '3050759'}, {'name': 'Makefile', 'bytes': '16052'}, {'name': 'PHP', 'bytes': '5703593'}, {'name': 'Perl', 'bytes': '50950'}, {'name': 'Shell', 'bytes': '27882'}, {'name': 'Smarty', 'bytes': '3929'}]} |
namespace MemeCentral.Server
{
using System;
using System.Linq;
using System.Web.ModelBinding;
using Microsoft.AspNet.Identity;
using Data.Models;
using Events;
using Controls;
using System.Collections.Generic;
using System.Web.UI.WebControls;
public partial class MemeDetails : BasePage
{
protected void Page_Load(object sender, EventArgs e)
{
}
// The id parameter should match the DataKeyNames value set on the control
// or be decorated with a value provider attribute, e.g. [QueryString]int id
public Meme MemeViewArticle_GetItem([QueryString("id")]int? id)
{
var isUserLogged = this.User.Identity.IsAuthenticated;
if (!isUserLogged)
{
this.Response.Redirect("/Account/Login");
}
var meme = this.dbContext.Memes.Where(x => x.Id == id).FirstOrDefault();
if (id == null | meme == null)
{
this.Response.Redirect("/NotFound");
}
this.CurrentUserId = meme.UserId;
return meme;
}
protected void CommentControl_Comment(object sender, CommentEventArgs e)
{
var userID = this.User.Identity.GetUserId();
var meme = this.dbContext.Memes.Find(e.DataID);
var comment = new Comment() { MemeId = meme.Id, UserId = userID, Content = e.Content, CreationDate = DateTime.Now };
meme.Comments.Add(comment);
this.dbContext.SaveChanges();
var control = sender as CommentControl;
control.Comments = meme.Comments.OrderByDescending(x => x.CreationDate).ToList();
}
protected List<Comment> GetComments(Meme item)
{
return item.Comments
.OrderByDescending(x => x.CreationDate)
.ToList();
}
protected int GetLikes(Meme item)
{
return item.Likes.Where(x => x.Value == true)
.ToList()
.Count();
}
protected int GetDislikes(Meme item)
{
return item.Likes.Where(x => x.Value == false)
.ToList()
.Count();
}
protected int HasUserVoted(Meme Item)
{
var userID = this.User.Identity.GetUserId();
var hasUserVoted = Item.Likes
.Where(x => x.UserId == userID)
.FirstOrDefault();
if (hasUserVoted == null)
{
return -1;
}
return 1;
}
protected void LikeControl_Like(object sender, LikeEventArgs e)
{
var userID = this.User.Identity.GetUserId();
var meme = this.dbContext.Memes.Find(e.DataID);
var rating = new Like();
rating.MemeId = e.DataID;
rating.UserId = userID;
if (e.LikeValue == 1)
{
rating.Value = true;
}
else
{
rating.Value = false;
}
meme.Likes.Add(rating);
this.dbContext.SaveChanges();
var control = sender as LikeControl;
control.Likes = this.GetLikes(meme);
control.Dislikes = this.GetDislikes(meme);
control.UserHasVoted = 1;
}
protected void DeleteButton_Command(object sender, CommandEventArgs e)
{
var memeId = e.CommandArgument.ToString();
var meme = this.dbContext.Memes.Find(int.Parse(memeId));
var likes = meme.Likes.ToArray();
var comments = meme.Comments.ToArray();
foreach (var like in likes)
{
this.dbContext.Likes.Remove(like);
}
foreach (var comment in comments)
{
this.dbContext.Comments.Remove(comment);
}
this.dbContext.Memes.Remove(meme);
var result = this.dbContext.SaveChanges();
if (result == 1)
{
this.Response.Redirect("/");
}
}
protected bool IsUserCreator()
{
var userID = this.User.Identity.GetUserId();
var isUserOwner = this.CurrentUserId == userID;
return isUserOwner;
}
private string CurrentUserId
{
get
{
if (ViewState["CurrentUserId"] != null)
{
return (string)(ViewState["CurrentUserId"]);
}
else
{
return "NaN";
}
}
set { ViewState["CurrentUserId"] = value; }
}
}
} | {'content_hash': '7215885c6884887694eccace33c15793', 'timestamp': '', 'source': 'github', 'line_count': 166, 'max_line_length': 128, 'avg_line_length': 28.41566265060241, 'alnum_prop': 0.517065931736273, 'repo_name': 'The-Last-Of-Us/MemeCentral', 'id': '7ab600d88aa454d09d65c05619b7a08ab796f19d', 'size': '4719', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'MemeCentral/MemeCentral.Server/MemeDetails.aspx.cs', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'ASP', 'bytes': '48580'}, {'name': 'C#', 'bytes': '76636'}, {'name': 'CSS', 'bytes': '2048'}, {'name': 'JavaScript', 'bytes': '170489'}]} |
A loading view animation
##Screenshots
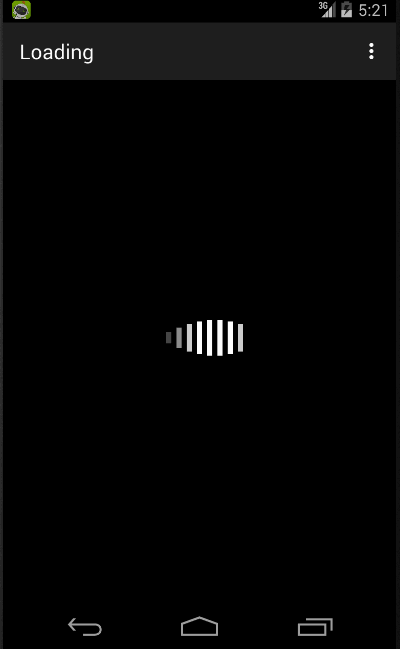
##
The MIT License (MIT)
Copyright (c) 2015 dodola
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE.
| {'content_hash': '20851853cb7dcdead7ce1577e642c00b', 'timestamp': '', 'source': 'github', 'line_count': 31, 'max_line_length': 91, 'avg_line_length': 39.12903225806452, 'alnum_prop': 0.798845836768343, 'repo_name': 'dodola/WaveLoadingView', 'id': '22c285c3e9722374d17cf2f90eaabd7d5f5b92ed', 'size': '1232', 'binary': False, 'copies': '2', 'ref': 'refs/heads/master', 'path': 'README.md', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Java', 'bytes': '5471'}]} |
#include "ChronoRage.h"
#include <Core/TGA.h>
#include <Core/System/System.h>
#include <Core/VFS/VFSXMLTranslator.h>
#include <Audio/AudioGlobals.h>
#include <Audio/AudioOpenAL/DriverOpenAL.h>
#include <GameSettings.h>
#include <Modes/GameModeDemo.h>
#include <Modes/GameModeLevels.h>
#include <Modes/GameModeTimeTrial.h>
#include <Modes/GameModeSurvival.h>
#include <Modes/GameModeDodge.h>
#include <Modes/GameModeRevenge.h>
#include <Modes/GameModeCredits.h>
#include <Modes/MenuMode.h>
#include <Modes/StartMode.h>
#include <Modes/GameMode1.h>
namespace ChronoRage
{
const bool TEST_MODE = false;
const String CHRONORAGE_GAME_SETTINGS_FILE = L"/User/Settings.xml";
const String CHRONORAGE_HIGH_SCORES_FILE = L"/User/HighScores.xml";
const String CHRONORAGE_LEVEL_RANKS_FILE = L"/ChronoRageLevels/level-ranks.xml";
const String CHRONORAGE_SURVIVAL_WAVE_FILE = L"/ChronoRageLevels/Waves/waves-survival.xml";
const String CHRONORAGE_DEMO_WAVE_FILE = L"/ChronoRageLevels/Waves/waves-demo.xml";
const String CHRONORAGE_DODGE_WAVE_FILE = L"/ChronoRageLevels/Waves/waves-dodge.xml";
ChronoRage::ChronoRage()
: _pGameState(null)
{
#ifdef LM_DEVMODE
_showRenderStats = false;
#endif
}
ChronoRage::~ChronoRage()
{
#if LM_DISPLAY_FPS
_pTextFPS = null;
_pTextFoesNumber = null;
_pTextPowerLevel = null;
_pTextTimeWarpNumber = null;
_pFontFPS = null;
_pHUD = null;
#endif
_pGameState = null;
///
_appContext.pSoundLibrary = null;
_appContext.pMenuMusic = null;
_appContext.pGameMusic = null;
_appContext.pSoundWorld = null;
_appContext.pAudioDevice = null;
_appContext.pAudioDriver = null;
///
_appContext.pCameraPivot = null;
_appContext.pCameraNode = null;
_appContext.pWorld = null;
///
_appContext.pVoxelFont1 = null;
_appContext.pVoxelFont2 = null;
_appContext.pVoxelCreditFont = null;
///
_appContext.pCursorArrow = null;
_appContext.pCursorReticle = null;
_appContext.pWidgetFactory = null;
if(_appContext.pGUI != null)
{
_appContext.pGUI->reset();
_appContext.pGUI = null;
}
_appContext.pRenderer = null;
_appContext.pDriver = null;
_appContext.pWinHandler = null;
_appContext.pInputManager = null;
_appContext.pRenderWindow = null;
_appContext.pVFS = null;
_appContext.pGameSettings = null;
_appContext.pHighScores = null;
_appContext.pLevelRanks = null;
_appContext.pDico = null;
}
bool ChronoRage::initialise()
{
#ifdef LM_DEVMODE
Core::String workDir(LM_WORKING_DIRECTORY);
SetCurrentDirectory(Core::String8(workDir).c_str());
#endif
INF << L"---------------------------------------------------------\n";
INF << L"System informations\n";
INF << L"---------------------------------------------------------\n";
INF << L"OS Version : " << Core::System::getOSName() << L"\n";
INF << L"CPU type : " << Core::System::getCPUName() << L"\n";
INF << L"CPU count : " << Core::toString(Core::System::getCPUCount()) << L"\n";
INF << L"Physical RAM : " << Core::toStringByteSize(Core::System::getTotalPhysicalRAM()) << L"\n";
INF << L"File swap RAM : " << Core::toStringByteSize(Core::System::getTotalFileSwapRAM()) << L"\n";
INF << L"Virtual RAM : " << Core::toStringByteSize(Core::System::getTotalVirtualRAM()) << L"\n";
INF << L"Current dir : " << Core::System::getCurrentDir() << L"\n";
INF << L"---------------------------------------------------------\n";
INF << L"Virtual file system layout\n";
INF << L"---------------------------------------------------------\n";
#ifdef LM_DEVMODE
// Initialisation VFS ---------------------------------------------
{
Ptr<Core::XMLDocument> pXmlDocument(new Core::XMLDocument());
if(pXmlDocument->loadDocument(L"./Data/VFS-ChronoRage.xml"))
{
_appContext.pVFS = Core::VFSFromXMLNode(pXmlDocument->firstChildNode(L"vfs"));
INF << _appContext.pVFS->toString() << "\n";
}
else
throw Core::Exception(L"Unable to mount VFS.");
}
#else
// Initialisation VFS ---------------------------------------------
{
Ptr<Core::XMLDocument> pXmlDocument(new Core::XMLDocument());
if(pXmlDocument->loadDocument(L"./VFS-ChronoRage.xml"))
{
_appContext.pVFS = Core::VFSFromXMLNode(pXmlDocument->firstChildNode(L"vfs"));
INF << _appContext.pVFS->toString() << "\n";
}
else
throw Core::Exception(L"Unable to mount VFS.");
}
#endif
// Dico load file
const Core::String DICO_FILE = L"/ChronoRageDictionaries/main.xml";
Ptr<Core::InputStream> pInputDic = _appContext.pVFS->readFile(DICO_FILE);
if(pInputDic == null)
{
Core::String message;
message << L"Unable to open dictionary file : " << DICO_FILE;
throw Core::Exception(message);
}
_appContext.pDriver = Gfx::IDriverPtr(new Gfx::DriverDx9());
Gfx::DisplayMode displayMode = _appContext.pDriver->getAdapterDisplayMode(0);
//Game Settings
bool specialFx = false;
Renderer::RendererSettings settings;
Renderer::ConfigProfile profile;
profile.msaaLevel = Renderer::MSAA_4X;
profile.minWidth = 1024;
profile.minHeight = 720;
profile.textureVRAMRatio = 0.7f;
// Auto config details
const Gfx::GraphicCapabilities & caps = _appContext.pDriver->getGraphicCaps(0);
if(int32(caps.psVersion) >= int32(Gfx::PS_V3_0))
{
if(caps.videoMem >= (400 << 20))
{
INF << L"Special effects should be turned *on* by default, MSAA 4X by default\n";
specialFx = true;
profile.msaaLevel = Renderer::MSAA_4X;
}
else if(caps.videoMem >= (200 << 20))
{
INF << L"Special effects should be turned *on* by default, MSAA NONE by default\n";
specialFx = true;
profile.msaaLevel = Renderer::MSAA_NONE;
}
else
{
INF << L"Special effects should be turned *off* by default, MSAA NONE by default\n";
specialFx = false;
profile.msaaLevel = Renderer::MSAA_NONE;
}
}
else if(int32(caps.psVersion) >= int32(Gfx::PS_V2_0))
{
INF << L"Special effects should be turned *off* by default, MSAA NONE by default\n";
specialFx = false;
profile.msaaLevel = Renderer::MSAA_NONE;
}
else
{
ERR << L"Video card does not support PS V2.0, exiting game with an error message\n";
Ptr<Core::Dictionary> pDico(new Core::Dictionary(Core::System::getSystemSupportedLanguage(), *pInputDic));
throw Core::Exception((*pDico)[L"bad-gpu"]);
}
Renderer::RendererSM2::autoConfig(_appContext.pDriver, 0, settings, profile);
Ptr<GameSettings> pGameSettings(new GameSettings(settings.width, settings.height, settings.msaaLevel));
pGameSettings->enableSpecialEffects(specialFx);
_appContext.pGameSettings = pGameSettings;
Ptr<Core::InputStream> pStreamSettingsFile = _appContext.pVFS->readFile(CHRONORAGE_GAME_SETTINGS_FILE);
if(pStreamSettingsFile != null)
{
Ptr<Core::XMLDocument> pXmlGameSettings(new Core::XMLDocument());
if(pXmlGameSettings->loadDocument(*pStreamSettingsFile))
_appContext.pGameSettings->importXML(pXmlGameSettings);
INF << L"Configuration loaded from file\n";
}
else
{
Ptr<Core::OutputStream> pSettingsOutput(_appContext.pVFS->writeFile(CHRONORAGE_GAME_SETTINGS_FILE));
if(pSettingsOutput != null)
{
Ptr<Core::XMLDocument> pXMLDoc(new Core::XMLDocument());
_appContext.pGameSettings->exportXML(pXMLDoc);
pXMLDoc->saveDocument(*pSettingsOutput);
pSettingsOutput->close();
}
INF << L"No configuration file found, using autoconfig setup\n";
}
//Ranks
Ptr<LevelRanks> pLevelRanks(new LevelRanks());
_appContext.pLevelRanks = pLevelRanks;
Ptr<Core::InputStream> pStreamLevelRanksFile = _appContext.pVFS->readFile(CHRONORAGE_LEVEL_RANKS_FILE);
if(pStreamLevelRanksFile != null)
{
Ptr<Core::XMLDocument> pXmlLevelRanks(new Core::XMLDocument());
if(pXmlLevelRanks->loadDocument(*pStreamLevelRanksFile))
_appContext.pLevelRanks->importXML(pXmlLevelRanks);
}
//High Scores
#ifndef CHRONORAGE_DEMO
Ptr<HighScores> pHighScores(new HighScores(pLevelRanks));
_appContext.pHighScores = pHighScores;
Ptr<Core::InputStream> pStreamHighScoresFile = _appContext.pVFS->readFile(CHRONORAGE_HIGH_SCORES_FILE);
if(pStreamHighScoresFile != null)
{
Ptr<Core::XMLDocument> pXmlHighScores(new Core::XMLDocument());
if(pXmlHighScores->loadDocument(*pStreamHighScoresFile))
_appContext.pHighScores->importXML(pXmlHighScores);
}
else
{
Ptr<Core::OutputStream> pHighScoresOutput(_appContext.pVFS->writeFile(CHRONORAGE_HIGH_SCORES_FILE));
if(pHighScoresOutput != null)
{
Ptr<Core::XMLDocument> pXMLDoc(new Core::XMLDocument());
_appContext.pHighScores->exportXML(pXMLDoc);
pXMLDoc->saveDocument(*pHighScoresOutput);
pHighScoresOutput->close();
}
}
#else
_appContext.pHighScores = null;
#endif
_appContext.exitState = DO_NOT_EXIT;
_appContext.saveScores = false;
_appContext.fastTitle = false;
HICON hMyIcon = LoadIcon(getInstance(), "IDI_ICON1");
_appContext.pInputManager = Ptr<Window::InputManager>(new Window::InputManager());
_appContext.pWinHandler = Ptr<Window::WinHandler>(new Window::WinHandler(_appContext.pInputManager));
_appContext.pRenderWindow = Ptr<Window::Window>(new Window::Window(
getInstance(),
L"Chrono Rage",
0, 0, // position
_appContext.pGameSettings->getScreenWidth(), // width
_appContext.pGameSettings->getScreenHeight(), // height
_appContext.pGameSettings->fullscreen(), // fullscreen
_appContext.pWinHandler,
hMyIcon));
_appContext.pInputManager->initialise(_appContext.pRenderWindow->getHandle());
// Pour éviter de référencer un joystick qui n'est plus branché
_appContext.pGameSettings->cleanupSettings(*_appContext.pInputManager);
_appContext.pRenderer = Ptr<Renderer::IRenderer>(new Renderer::RendererSM2(
_appContext.pDriver,
_appContext.pGameSettings->getRendererSettings(),
_appContext.pRenderWindow->getHandle(),
_appContext.pVFS,
L"/Shaders"));
Audio::initVorbisFileDll();
_appContext.pAudioDriver = Ptr<Audio::IDriver>(new AudioOpenAL::DriverOpenAL());
_appContext.pAudioDevice = _appContext.pAudioDriver->createDevice(0);
_appContext.pSoundLibrary = Ptr<SoundLibrary>(new SoundLibrary(_appContext));
bool result = _appContext.pRenderer->initialise();
_appContext.pWorld = Ptr<Universe::World>(new Universe::World(_appContext.pRenderer, _appContext.pVFS, null, null));
_appContext.pSoundWorld = Ptr<Universe::World>(new Universe::World(_appContext.pRenderer, _appContext.pVFS, null, _appContext.pAudioDevice));
_appContext.pMenuMusic = null;
_appContext.pGameMusic = null;
Ptr<Universe::NodeListener> pNodeListener = _appContext.pSoundWorld->getNodeListener();
if(pNodeListener != null)
pNodeListener->setGain(1.0f);
_appContext.pGUI = Ptr<GUI::Manager>(new GUI::Manager(_appContext.pVFS, _appContext.pRenderWindow, _appContext.pInputManager, _appContext.pRenderer, _appContext.pWorld->getRessourcesPool()));
_appContext.pGUI->getResPool()->mountPictureBank(L"/ChronoRageData/GUI", L"/ChronoRageData/GUI.pbk");
#ifdef CHRONORAGE_DEMO
_appContext.pGUI->getResPool()->mountPictureBank(L"/ChronoRageData/DemoGUI", L"/ChronoRageData/DemoGUI.pbk");
#endif
_appContext.pCursorArrow = Core::loadTGA(*_appContext.pVFS->readFile(L"/ChronoRageData/arrow.tga"));
_appContext.pCursorReticle = Core::loadTGA(*_appContext.pVFS->readFile(L"/ChronoRageData/reticle.tga"));
_appContext.pCursorBlank = Core::loadTGA(*_appContext.pVFS->readFile(L"/ChronoRageData/blank.tga"));
_appContext.reloadDictionary = false;
_appContext.pDico = Ptr<Core::Dictionary>(new Core::Dictionary(pGameSettings->getLanguage(), *pInputDic));
_appContext.pWidgetFactory = Ptr<WidgetFactory>(new WidgetFactory( _appContext.pGUI,
_appContext.pInputManager,
_appContext.pDico,
_appContext.pSoundLibrary));
//_appContext.wantedMode = _currentMode = MENU_MODE;
_appContext.wantedMode = _currentMode = START_MODE;
_appContext.wantedScreen = MAIN_MENU_SCREEN;
if(result)
{
if(TEST_MODE)
{
Ptr<GameModeTimeTrial> pGameModeTimeTrial(new GameModeTimeTrial(_appContext));
_pGameState = pGameModeTimeTrial;
_pGameState->initialize();
pGameModeTimeTrial->setLevel(19);
/*Ptr<GameModeDemo> pGameModeDemo(new GameModeDemo(_appContext));
_pGameState = pGameModeDemo;
_pGameState->initialize();
pGameModeDemo->registerWaveFile(CHRONORAGE_DEMO_WAVE_FILE);*/
}
else
initGameState();
}
#if LM_DISPLAY_FPS
// Compteur FPS
_pHUD = _appContext.pRenderer->createHUD();
_pFontFPS = _appContext.pRenderer->createFont(L"Arial", 10);
_pTextFPS = _pHUD->createText(_pFontFPS, L"");
_pTextFPS->setColor(Core::Vector4f(0.7f, 0.7f, 0.7f, 1.0f));
_pTextFPS->setPosition(Core::Vector2f(5.0f, 5.0f));
_pTextFoesNumber = _pHUD->createText(_pFontFPS, L"");
_pTextFoesNumber->setColor(Core::Vector4f(1.0f, 0.7f, 0.7f, 1.0f));
_pTextFoesNumber->setPosition(Core::Vector2f(100.0f, 5.0f));
_pTextTimeWarpNumber = _pHUD->createText(_pFontFPS, L"");
_pTextTimeWarpNumber->setColor(Core::Vector4f(0.1f, 1.0f, 1.0f, 1.0f));
_pTextTimeWarpNumber->setPosition(Core::Vector2f(5.0f, 40.0f));
_pTextPowerLevel = _pHUD->createText(_pFontFPS, L"");
_pTextPowerLevel->setColor(Core::Vector4f(0.7f, 0.1f, 1.0f, 1.0f));
_pTextPowerLevel->setPosition(Core::Vector2f(5.0f, 60.0f));
#endif
return result;
}
void ChronoRage::run()
{
try
{
Application::run();
}
catch(Gfx::GfxException & exception)
{
ERR << L"Fatal GFX exception caught : " << exception.getMessage() << L"\n";
#ifdef _DEBUG
ERR << exception.getCallStack() << L"\n";
#endif
String error(L"A fatal error occurred in display module");
if(_appContext.pDico != null)
error = (*_appContext.pDico)[L"gfx-error"];
throw Core::Exception(error);
}
catch(Renderer::RendererException & exception)
{
ERR << L"Fatal GFX exception caught : " << exception.getMessage() << L"\n";
#ifdef _DEBUG
ERR << exception.getCallStack() << L"\n";
#endif
String error(L"A fatal error occurred in display module");
if(_appContext.pDico != null)
error = (*_appContext.pDico)[L"gfx-error"];
throw Core::Exception(error);
}
}
void ChronoRage::release()
{
//Sauvegarde settings
Ptr<Core::OutputStream> pSettingsOutput(_appContext.pVFS->writeFile(CHRONORAGE_GAME_SETTINGS_FILE));
if(pSettingsOutput != null)
{
Ptr<Core::XMLDocument> pXMLDoc(new Core::XMLDocument());
_appContext.pGameSettings->exportXML(pXMLDoc);
pXMLDoc->saveDocument(*pSettingsOutput);
pSettingsOutput->close();
}
#ifndef CHRONORAGE_DEMO
//Sauvegarde High Scores
Ptr<Core::OutputStream> pHighScoresOutput(_appContext.pVFS->writeFile(CHRONORAGE_HIGH_SCORES_FILE));
if(pHighScoresOutput != null)
{
Ptr<Core::XMLDocument> pXMLDoc(new Core::XMLDocument());
_appContext.pHighScores->exportXML(pXMLDoc);
pXMLDoc->saveDocument(*pHighScoresOutput);
pHighScoresOutput->close();
}
#endif
if(_appContext.pGameMusic != null)
{
_appContext.pGameMusic->kill();
_appContext.pGameMusic = null;
}
if(_appContext.pMenuMusic != null)
{
_appContext.pMenuMusic->kill();
_appContext.pMenuMusic = null;
}
Audio::shutdownVorbisFileDll();
}
void ChronoRage::update(double elapsed)
{
if(_appContext.reloadDictionary)
{
const Core::String DICO_FILE = L"/ChronoRageDictionaries/main.xml";
Ptr<Core::InputStream> pInputDic = _appContext.pVFS->readFile(DICO_FILE);
if(pInputDic == null)
{
Core::String message;
message << L"Unable to open dictionary file : " << DICO_FILE;
throw Core::Exception(message);
}
_appContext.reloadDictionary = false;
_appContext.pDico = Ptr<Core::Dictionary>(new Core::Dictionary(_appContext.pGameSettings->getLanguage(), *pInputDic));
_appContext.pWidgetFactory = Ptr<WidgetFactory>(new WidgetFactory( _appContext.pGUI,
_appContext.pInputManager,
_appContext.pDico,
_appContext.pSoundLibrary));
if(_pGameState->getMode() == MENU_MODE)
{
Ptr<MenuMode> pMenuMode = LM_DEBUG_PTR_CAST<MenuMode>(_pGameState);
pMenuMode->resetScreenLabels();
}
_appContext.reloadDictionary = false;
}
if(_appContext.wantedMode != _currentMode)
{
_currentMode = _appContext.wantedMode;
initGameState();
}
#ifndef CHRONORAGE_DEMO
if(_appContext.saveScores)
{
//Sauvegarde High Scores
Ptr<Core::OutputStream> pHighScoresOutput(_appContext.pVFS->writeFile(CHRONORAGE_HIGH_SCORES_FILE));
if(pHighScoresOutput != null)
{
Ptr<Core::XMLDocument> pXMLDoc(new Core::XMLDocument());
_appContext.pHighScores->exportXML(pXMLDoc);
pXMLDoc->saveDocument(*pHighScoresOutput);
pHighScoresOutput->close();
}
_appContext.saveScores = false;
}
#endif
if(_appContext.exitState == MUST_EXIT_NOW)
{
PostQuitMessage(0);
}
LM_ASSERT(_appContext.pSoundWorld != null);
_appContext.pSoundWorld->update(elapsed);
_pGameState->update(elapsed);
#ifdef LM_DEVMODE
if(_appContext.pInputManager->isKeyTyped(VK_ADD))
_pGameState->powerUp();
if(_appContext.pInputManager->isKeyTyped(VK_SUBTRACT))
_pGameState->powerDown();
int32 foeId = -1;
if(_appContext.pInputManager->isKeyTyped(VK_NUMPAD0))
foeId = 0;
if(_appContext.pInputManager->isKeyTyped(VK_NUMPAD1))
foeId = 1;
if(_appContext.pInputManager->isKeyTyped(VK_NUMPAD2))
foeId = 2;
if(_appContext.pInputManager->isKeyTyped(VK_NUMPAD3))
foeId = 3;
if(_appContext.pInputManager->isKeyTyped(VK_NUMPAD4))
foeId = 4;
if(_appContext.pInputManager->isKeyTyped(VK_NUMPAD5))
foeId = 5;
if(_appContext.pInputManager->isKeyTyped(VK_NUMPAD6))
foeId = 6;
if(_appContext.pInputManager->isKeyTyped(VK_NUMPAD7))
foeId = 7;
if(_appContext.pInputManager->isKeyTyped(VK_NUMPAD8))
foeId = 8;
if(_appContext.pInputManager->isKeyTyped(VK_NUMPAD9))
foeId = 9;
if(foeId >= 0)
_pGameState->createFoe(foeId);
if(_appContext.pInputManager->isKeyTyped(VK_F11))
_showRenderStats = !_showRenderStats;
if(_appContext.pInputManager->isKeyTyped(VK_F12))
Core::Allocator::getInstance().dumpMemoryUsage();
#endif/*LM_DEVMODE*/
}
void ChronoRage::render()
{
Ptr<Renderer::IRenderView> pView(_appContext.pRenderer->getDefaultView());
pView->resize(_appContext.pWinHandler->getWindowWidth(), _appContext.pWinHandler->getWindowHeight());
_pGameState->render(pView);
#if LM_DISPLAY_FPS
_fps.nextFrame();
if(_showRenderStats)
_pTextFPS->setText(_fps.getText() + L"\n\n\n\n\n" + _appContext.pRenderer->getRenderStats());
else
_pTextFPS->setText(_fps.getText());
Core::String foesNumber = L"Foes number : ";
foesNumber << _pGameState->getFoesNumber()
<< L" Nodes count : "
<< Core::toString(_appContext.pWorld->getAllNodes().size());
_pTextFoesNumber->setText(foesNumber);
Core::String timeWarpNumber = L"TimeWarp number : ";
timeWarpNumber << Core::toString(_pGameState->getTimeWarpNumber(), 2);
_pTextTimeWarpNumber->setText(timeWarpNumber);
Core::String powerLevel = L"Player Power Level : ";
powerLevel << Core::toString(_pGameState->getPowerLevel(), 2);
_pTextPowerLevel->setText(powerLevel);
//_appContext.pRenderer->renderHUD(pView, _pHUD);
#endif
pView->present();
}
void ChronoRage::initGameState()
{
try
{
switch(_currentMode)
{
case START_MODE:
_pGameState = Ptr<StartMode>(new StartMode(_appContext));
_pGameState->initialize();
break;
case MENU_MODE:
_pGameState = Ptr<MenuMode>(new MenuMode(_appContext));
_pGameState->initialize();
break;
#ifndef CHRONORAGE_DEMO
case NEW_GAME_MODE:
{
Ptr<GameModeLevels> pGameModeLevels(new GameModeLevels(_appContext));
_pGameState = pGameModeLevels;
_pGameState->initialize();
pGameModeLevels->setLevel(1);
}
break;
case CONTINUE_GAME_MODE:
{
Ptr<GameModeLevels> pGameModeLevels(new GameModeLevels(_appContext));
_pGameState = pGameModeLevels;
_pGameState->initialize();
pGameModeLevels->setLevel(_appContext.wantedLevel);
}
break;
case TIME_TRIAL_MODE:
{
Ptr<GameModeTimeTrial> pGameModeTimeTrial(new GameModeTimeTrial(_appContext));
_pGameState = pGameModeTimeTrial;
_pGameState->initialize();
pGameModeTimeTrial->setLevel(_appContext.wantedLevel);
}
break;
case SURVIVAL_MODE:
{
Ptr<GameModeSurvival> pGameModeSurvival(new GameModeSurvival(_appContext, false));
_pGameState = pGameModeSurvival;
_pGameState->initialize();
pGameModeSurvival->registerWaveFile(CHRONORAGE_SURVIVAL_WAVE_FILE);
}
break;
case SURVIVAL_HARD_MODE:
{
Ptr<GameModeSurvival> pGameModeSurvival(new GameModeSurvival(_appContext, true));
_pGameState = pGameModeSurvival;
_pGameState->initialize();
pGameModeSurvival->registerWaveFile(CHRONORAGE_SURVIVAL_WAVE_FILE);
}
break;
case DODGE_MODE:
{
Ptr<GameModeDodge> pGameModeDodge(new GameModeDodge(_appContext));
_pGameState = pGameModeDodge;
_pGameState->initialize();
pGameModeDodge->registerWaveFile(CHRONORAGE_DODGE_WAVE_FILE);
}
break;
case REVENGE_MODE:
{
Ptr<GameModeRevenge> pGameModeRevenge(new GameModeRevenge(_appContext));
_pGameState = pGameModeRevenge;
_pGameState->initialize();
pGameModeRevenge->registerWaveFile(CHRONORAGE_SURVIVAL_WAVE_FILE);
}
break;
#endif
case DEMO_MODE:
{
Ptr<GameModeDemo> pGameModeDemo(new GameModeDemo(_appContext));
_pGameState = pGameModeDemo;
_pGameState->initialize();
pGameModeDemo->registerWaveFile(CHRONORAGE_DEMO_WAVE_FILE);
}
break;
#ifndef CHRONORAGE_DEMO
case CREDITS_MODE:
{
Ptr<GameModeCredits> pGameModeCredits(new GameModeCredits(_appContext));
_pGameState = pGameModeCredits;
_pGameState->initialize();
pGameModeCredits->setLevel(_appContext.wantedLevel);
}
break;
#endif
}
}
catch(Core::Exception & e)
{
Core::System::errorMessageBox(e.getMessage());
ERR << L"Error initialising world : " << e.getMessage() << L"\n";
ERR << e.getCallStack();
}
}
}//namespace ChronoRage | {'content_hash': '460268b93d5216cd05638932eb9d7ee9', 'timestamp': '', 'source': 'github', 'line_count': 687, 'max_line_length': 195, 'avg_line_length': 37.15574963609898, 'alnum_prop': 0.5997414401002898, 'repo_name': 'benkaraban/anima-games-engine', 'id': 'b2792d88366c3c2beb8a919e642ccb3fe7a88e82', 'size': '27195', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'Sources/ChronoRage/ChronoRage.cpp', 'mode': '33188', 'license': 'bsd-3-clause', 'language': [{'name': 'C', 'bytes': '8772269'}, {'name': 'C#', 'bytes': '125904'}, {'name': 'C++', 'bytes': '74431764'}, {'name': 'CMake', 'bytes': '43555'}, {'name': 'Java', 'bytes': '95806'}, {'name': 'Makefile', 'bytes': '17914'}, {'name': 'NSIS', 'bytes': '17188'}, {'name': 'Objective-C', 'bytes': '50195'}, {'name': 'Perl', 'bytes': '6275'}, {'name': 'Python', 'bytes': '22678'}, {'name': 'Shell', 'bytes': '21728'}]} |
package org.apache.hadoop.hdfs.server.datanode;
import static org.apache.hadoop.hdfs.DFSConfigKeys.DFS_DATANODE_BALANCE_MAX_NUM_CONCURRENT_MOVES_KEY;
import static org.apache.hadoop.hdfs.DFSConfigKeys.DFS_DATANODE_BALANCE_MAX_NUM_CONCURRENT_MOVES_DEFAULT;
import static org.junit.Assert.assertEquals;
import static org.junit.Assert.assertTrue;
import static org.junit.Assert.fail;
import java.io.File;
import java.io.IOException;
import java.net.InetSocketAddress;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.conf.ReconfigurationException;
import org.apache.hadoop.fs.CommonConfigurationKeys;
import org.apache.hadoop.fs.FileUtil;
import org.apache.hadoop.hdfs.DFSConfigKeys;
import org.apache.hadoop.hdfs.HdfsConfiguration;
import org.apache.hadoop.hdfs.MiniDFSCluster;
import org.apache.hadoop.hdfs.MiniDFSNNTopology;
import org.junit.After;
import org.junit.Assert;
import org.junit.Before;
import org.junit.Test;
/**
* Test to reconfigure some parameters for DataNode without restart
*/
public class TestDataNodeReconfiguration {
private static final String DATA_DIR = MiniDFSCluster.getBaseDirectory()
+ "data";
private final static InetSocketAddress NN_ADDR = new InetSocketAddress(
"localhost", 5020);
private final int NUM_NAME_NODE = 1;
private final int NUM_DATA_NODE = 10;
private MiniDFSCluster cluster;
@Before
public void Setup() throws IOException {
startDFSCluster(NUM_NAME_NODE, NUM_DATA_NODE);
}
@After
public void tearDown() throws Exception {
if (cluster != null) {
cluster.shutdown();
cluster = null;
}
File dir = new File(DATA_DIR);
if (dir.exists())
Assert.assertTrue("Cannot delete data-node dirs",
FileUtil.fullyDelete(dir));
}
private void startDFSCluster(int numNameNodes, int numDataNodes)
throws IOException {
Configuration conf = new Configuration();
MiniDFSNNTopology nnTopology = MiniDFSNNTopology
.simpleFederatedTopology(numNameNodes);
cluster = new MiniDFSCluster.Builder(conf).nnTopology(nnTopology)
.numDataNodes(numDataNodes).build();
cluster.waitActive();
}
/**
* Starts an instance of DataNode
*
* @throws IOException
*/
public DataNode[] createDNsForTest(int numDateNode) throws IOException {
Configuration conf = new HdfsConfiguration();
conf.set(DFSConfigKeys.DFS_DATANODE_DATA_DIR_KEY, DATA_DIR);
conf.set(DFSConfigKeys.DFS_DATANODE_ADDRESS_KEY, "0.0.0.0:0");
conf.set(DFSConfigKeys.DFS_DATANODE_HTTP_ADDRESS_KEY, "0.0.0.0:0");
conf.set(DFSConfigKeys.DFS_DATANODE_IPC_ADDRESS_KEY, "0.0.0.0:0");
conf.setInt(CommonConfigurationKeys.IPC_CLIENT_CONNECT_MAX_RETRIES_KEY, 0);
DataNode[] result = new DataNode[numDateNode];
for (int i = 0; i < numDateNode; i++) {
result[i] = InternalDataNodeTestUtils.startDNWithMockNN(conf, NN_ADDR, DATA_DIR);
}
return result;
}
@Test
public void testMaxConcurrentMoversReconfiguration()
throws ReconfigurationException, IOException {
int maxConcurrentMovers = 10;
for (int i = 0; i < NUM_DATA_NODE; i++) {
DataNode dn = cluster.getDataNodes().get(i);
// try invalid values
try {
dn.reconfigureProperty(
DFS_DATANODE_BALANCE_MAX_NUM_CONCURRENT_MOVES_KEY, "text");
fail("ReconfigurationException expected");
} catch (ReconfigurationException expected) {
assertTrue("expecting NumberFormatException",
expected.getCause() instanceof NumberFormatException);
}
try {
dn.reconfigureProperty(
DFS_DATANODE_BALANCE_MAX_NUM_CONCURRENT_MOVES_KEY,
String.valueOf(-1));
fail("ReconfigurationException expected");
} catch (ReconfigurationException expected) {
assertTrue("expecting IllegalArgumentException",
expected.getCause() instanceof IllegalArgumentException);
}
try {
dn.reconfigureProperty(
DFS_DATANODE_BALANCE_MAX_NUM_CONCURRENT_MOVES_KEY,
String.valueOf(0));
fail("ReconfigurationException expected");
} catch (ReconfigurationException expected) {
assertTrue("expecting IllegalArgumentException",
expected.getCause() instanceof IllegalArgumentException);
}
// change properties
dn.reconfigureProperty(DFS_DATANODE_BALANCE_MAX_NUM_CONCURRENT_MOVES_KEY,
String.valueOf(maxConcurrentMovers));
// verify change
assertEquals(String.format("%s has wrong value",
DFS_DATANODE_BALANCE_MAX_NUM_CONCURRENT_MOVES_KEY),
maxConcurrentMovers, dn.xserver.balanceThrottler.getMaxConcurrentMovers());
assertEquals(String.format("%s has wrong value",
DFS_DATANODE_BALANCE_MAX_NUM_CONCURRENT_MOVES_KEY),
maxConcurrentMovers, Integer.parseInt(dn.getConf().get(
DFS_DATANODE_BALANCE_MAX_NUM_CONCURRENT_MOVES_KEY)));
// revert to default
dn.reconfigureProperty(DFS_DATANODE_BALANCE_MAX_NUM_CONCURRENT_MOVES_KEY,
null);
// verify default
assertEquals(String.format("%s has wrong value",
DFS_DATANODE_BALANCE_MAX_NUM_CONCURRENT_MOVES_KEY),
DFS_DATANODE_BALANCE_MAX_NUM_CONCURRENT_MOVES_DEFAULT,
dn.xserver.balanceThrottler.getMaxConcurrentMovers());
assertEquals(String.format("expect %s is not configured",
DFS_DATANODE_BALANCE_MAX_NUM_CONCURRENT_MOVES_KEY), null, dn
.getConf().get(DFS_DATANODE_BALANCE_MAX_NUM_CONCURRENT_MOVES_KEY));
}
}
@Test
public void testAcquireWithMaxConcurrentMoversGreaterThanDefault()
throws IOException, ReconfigurationException {
final DataNode[] dns = createDNsForTest(1);
try {
testAcquireOnMaxConcurrentMoversReconfiguration(dns[0], 10);
} finally {
dns[0].shutdown();
}
}
@Test
public void testAcquireWithMaxConcurrentMoversLessThanDefault()
throws IOException, ReconfigurationException {
final DataNode[] dns = createDNsForTest(1);
try {
testAcquireOnMaxConcurrentMoversReconfiguration(dns[0], 3);
} finally {
dns[0].shutdown();
}
}
/**
* Simulates a scenario where the DataNode has been reconfigured with fewer
* mover threads, but all of the current treads are busy and therefore the
* DataNode is unable to honor this request within a reasonable amount of
* time. The DataNode eventually gives up and returns a flag indicating that
* the request was not honored.
*/
@Test
public void testFailedDecreaseConcurrentMovers()
throws IOException, ReconfigurationException {
final DataNode[] dns = createDNsForTest(1);
final DataNode dataNode = dns[0];
try {
// Set the current max to 2
dataNode.xserver.updateBalancerMaxConcurrentMovers(2);
// Simulate grabbing 2 threads
dataNode.xserver.balanceThrottler.acquire();
dataNode.xserver.balanceThrottler.acquire();
dataNode.xserver.setMaxReconfigureWaitTime(1);
// Attempt to set new maximum to 1
final boolean success =
dataNode.xserver.updateBalancerMaxConcurrentMovers(1);
Assert.assertFalse(success);
} finally {
dataNode.shutdown();
}
}
/**
* Test with invalid configuration.
*/
@Test(expected = ReconfigurationException.class)
public void testFailedDecreaseConcurrentMoversReconfiguration()
throws IOException, ReconfigurationException {
final DataNode[] dns = createDNsForTest(1);
final DataNode dataNode = dns[0];
try {
// Set the current max to 2
dataNode.xserver.updateBalancerMaxConcurrentMovers(2);
// Simulate grabbing 2 threads
dataNode.xserver.balanceThrottler.acquire();
dataNode.xserver.balanceThrottler.acquire();
dataNode.xserver.setMaxReconfigureWaitTime(1);
// Now try reconfigure maximum downwards with threads released
dataNode.reconfigurePropertyImpl(
DFS_DATANODE_BALANCE_MAX_NUM_CONCURRENT_MOVES_KEY, "1");
} catch (ReconfigurationException e) {
Assert.assertEquals(DFS_DATANODE_BALANCE_MAX_NUM_CONCURRENT_MOVES_KEY,
e.getProperty());
Assert.assertEquals("1", e.getNewValue());
throw e;
} finally {
dataNode.shutdown();
}
}
private void testAcquireOnMaxConcurrentMoversReconfiguration(
DataNode dataNode, int maxConcurrentMovers) throws IOException,
ReconfigurationException {
final int defaultMaxThreads = dataNode.getConf().getInt(
DFSConfigKeys.DFS_DATANODE_BALANCE_MAX_NUM_CONCURRENT_MOVES_KEY,
DFSConfigKeys.DFS_DATANODE_BALANCE_MAX_NUM_CONCURRENT_MOVES_DEFAULT);
/** Test that the default setup is working */
for (int i = 0; i < defaultMaxThreads; i++) {
assertEquals("should be able to get thread quota", true,
dataNode.xserver.balanceThrottler.acquire());
}
assertEquals("should not be able to get thread quota", false,
dataNode.xserver.balanceThrottler.acquire());
// Give back the threads
for (int i = 0; i < defaultMaxThreads; i++) {
dataNode.xserver.balanceThrottler.release();
}
/** Test that the change is applied correctly */
// change properties
dataNode.reconfigureProperty(
DFS_DATANODE_BALANCE_MAX_NUM_CONCURRENT_MOVES_KEY,
String.valueOf(maxConcurrentMovers));
assertEquals("thread quota is wrong", maxConcurrentMovers,
dataNode.xserver.balanceThrottler.getMaxConcurrentMovers());
for (int i = 0; i < maxConcurrentMovers; i++) {
assertEquals("should be able to get thread quota", true,
dataNode.xserver.balanceThrottler.acquire());
}
assertEquals("should not be able to get thread quota", false,
dataNode.xserver.balanceThrottler.acquire());
}
}
| {'content_hash': 'af0bf60b53dd08f9c225738c3c963063', 'timestamp': '', 'source': 'github', 'line_count': 280, 'max_line_length': 105, 'avg_line_length': 35.10357142857143, 'alnum_prop': 0.7024112320683691, 'repo_name': 'apurtell/hadoop', 'id': '8cbd38bc601dcee68e218e840b489e8bdfe8f9c4', 'size': '10635', 'binary': False, 'copies': '2', 'ref': 'refs/heads/trunk', 'path': 'hadoop-hdfs-project/hadoop-hdfs/src/test/java/org/apache/hadoop/hdfs/server/datanode/TestDataNodeReconfiguration.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Batchfile', 'bytes': '78445'}, {'name': 'C', 'bytes': '2023750'}, {'name': 'C++', 'bytes': '2873786'}, {'name': 'CMake', 'bytes': '120569'}, {'name': 'CSS', 'bytes': '94990'}, {'name': 'Dockerfile', 'bytes': '4613'}, {'name': 'HTML', 'bytes': '221057'}, {'name': 'Handlebars', 'bytes': '207062'}, {'name': 'Java', 'bytes': '97177756'}, {'name': 'JavaScript', 'bytes': '1273196'}, {'name': 'Python', 'bytes': '14938'}, {'name': 'SCSS', 'bytes': '23607'}, {'name': 'Shell', 'bytes': '517782'}, {'name': 'TLA', 'bytes': '14997'}, {'name': 'TSQL', 'bytes': '17801'}, {'name': 'TeX', 'bytes': '19322'}, {'name': 'XSLT', 'bytes': '18026'}]} |
<!doctype html>
<!-- This file is generated by build.py. -->
<title>video tall.jpg; overflow:hidden; -o-object-fit:contain; -o-object-position:30px bottom</title>
<link rel="stylesheet" href="../../support/reftests.css">
<link rel='match' href='hidden_contain_30px_bottom-ref.html'>
<style>
#test > * { overflow:hidden; -o-object-fit:contain; -o-object-position:30px bottom }
</style>
<div id="test">
<video poster="../../support/tall.jpg"></video>
</div>
| {'content_hash': '808f658da6eff603c47f393afac61a2b', 'timestamp': '', 'source': 'github', 'line_count': 11, 'max_line_length': 101, 'avg_line_length': 41.63636363636363, 'alnum_prop': 0.6855895196506551, 'repo_name': 'Ms2ger/presto-testo', 'id': '8049bf4ad6f21303cb4f8e689871ca2a039027a0', 'size': '458', 'binary': False, 'copies': '4', 'ref': 'refs/heads/master', 'path': 'css/image-fit/reftests/video-poster-jpg-tall/hidden_contain_30px_bottom.html', 'mode': '33188', 'license': 'bsd-3-clause', 'language': [{'name': 'ASP', 'bytes': '2312'}, {'name': 'ActionScript', 'bytes': '23470'}, {'name': 'AutoHotkey', 'bytes': '8832'}, {'name': 'Batchfile', 'bytes': '5001'}, {'name': 'C', 'bytes': '116512'}, {'name': 'C++', 'bytes': '219233'}, {'name': 'CSS', 'bytes': '207914'}, {'name': 'Erlang', 'bytes': '18523'}, {'name': 'Groff', 'bytes': '674'}, {'name': 'HTML', 'bytes': '103272540'}, {'name': 'Haxe', 'bytes': '3874'}, {'name': 'Java', 'bytes': '125658'}, {'name': 'JavaScript', 'bytes': '22516936'}, {'name': 'Makefile', 'bytes': '13409'}, {'name': 'PHP', 'bytes': '524911'}, {'name': 'Perl', 'bytes': '321672'}, {'name': 'Python', 'bytes': '948191'}, {'name': 'Ruby', 'bytes': '1006850'}, {'name': 'Shell', 'bytes': '12140'}, {'name': 'Smarty', 'bytes': '1860'}, {'name': 'XSLT', 'bytes': '2567445'}]} |
require 'shell_shock/context'
require 'cardigan/commands'
module Cardigan
class EntryContext
include ShellShock::Context
def initialize io, workflow_repository, entry
@io, @workflow_repository, @entry = io, workflow_repository, entry
@prompt_text = "#{File.expand_path('.').split('/').last.slice(0..0)}/#{entry['name']} > "
@commands = {
'now' => Command.load(:change_status, @entry, @workflow_repository),
'set' => Command.load(:change_value, @entry, @io),
'edit' => Command.load(:edit_value, @entry, @io),
'ls' => Command.load(:show_entry, @entry, @io)
}
end
end
end | {'content_hash': '0ca59fcad93e42a9e20083e80e07337f', 'timestamp': '', 'source': 'github', 'line_count': 19, 'max_line_length': 95, 'avg_line_length': 33.89473684210526, 'alnum_prop': 0.6164596273291926, 'repo_name': 'markryall/cardigan', 'id': 'd2c5590562e4fd5300927c3857812467621978ce', 'size': '644', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'lib/cardigan/entry_context.rb', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Ruby', 'bytes': '40805'}]} |
package com.eusecom.attendance.fragment;
import android.app.Dialog;
import android.app.ProgressDialog;
import android.content.Intent;
import android.os.Bundle;
import android.support.v4.app.Fragment;
import android.support.v7.widget.LinearLayoutManager;
import android.support.v7.widget.RecyclerView;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.TextView;
import android.widget.Toast;
import com.eusecom.attendance.NewPostActivity;
import com.eusecom.attendance.SettingsActivity;
import com.eusecom.attendance.models.Attendance;
import com.eusecom.attendance.models.DeletedAbs;
import com.firebase.ui.database.FirebaseRecyclerAdapter;
import com.google.firebase.auth.FirebaseAuth;
import com.google.firebase.database.DataSnapshot;
import com.google.firebase.database.DatabaseError;
import com.google.firebase.database.DatabaseReference;
import com.google.firebase.database.FirebaseDatabase;
import com.google.firebase.database.MutableData;
import com.google.firebase.database.Query;
import com.google.firebase.database.ServerValue;
import com.google.firebase.database.Transaction;
import com.eusecom.attendance.R;
import com.eusecom.attendance.models.Post;
import com.eusecom.attendance.viewholder.AbsenceViewHolder;
import com.google.firebase.database.ValueEventListener;
import java.util.HashMap;
import java.util.Map;
public abstract class AbsenceListFragment extends Fragment {
private static final String TAG = "AbsenceListFragment";
// [START define_database_reference]
private DatabaseReference mDatabase;
// [END define_database_reference]
private FirebaseRecyclerAdapter<Attendance, AbsenceViewHolder> mAdapter;
private RecyclerView mRecycler;
private LinearLayoutManager mManager;
public AbsenceListFragment() {}
String absxy;
String abskeydel=null;
private ProgressDialog fProgressDialog;
boolean isCancelable, isrunning;
String timestampx;
@Override
public View onCreateView (LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
super.onCreateView(inflater, container, savedInstanceState);
View rootView = inflater.inflate(R.layout.fragment_absences, container, false);
// [START create_database_reference]
mDatabase = FirebaseDatabase.getInstance().getReference();
// [END create_database_reference]
mRecycler = (RecyclerView) rootView.findViewById(R.id.absences_list);
mRecycler.setHasFixedSize(true);
return rootView;
}
@Override
public void onActivityCreated(Bundle savedInstanceState) {
super.onActivityCreated(savedInstanceState);
showfProgressDialog();
DatabaseReference connectedRef = FirebaseDatabase.getInstance().getReference(".info/connected");
connectedRef.addValueEventListener(new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot snapshot) {
boolean connected = snapshot.getValue(Boolean.class);
if (connected) {
System.out.println("connected");
if(isrunning) { showfProgressDialog(); }
} else {
System.out.println("not connected");
hidefProgressDialog();
if(isrunning) { Toast.makeText(getActivity(), getResources().getString(R.string.notconnected), Toast.LENGTH_SHORT).show(); }
}
}
@Override
public void onCancelled(DatabaseError error) {
System.err.println("Listener was cancelled");
}
});
DatabaseReference gettimestramp = FirebaseDatabase.getInstance().getReference("gettimestamp");
gettimestramp.addValueEventListener(new ValueEventListener() {
public void onDataChange(DataSnapshot dataSnapshot) {
//System.out.println(dataSnapshot.getValue());
timestampx=dataSnapshot.getValue().toString();
//Log.d(TAG, "ServerValue.TIMESTAMP " + timestampx);
}
public void onCancelled(DatabaseError databaseError) { }
});
gettimestramp.setValue(ServerValue.TIMESTAMP);
// Set up Layout Manager, reverse layout
mManager = new LinearLayoutManager(getActivity());
mManager.setReverseLayout(true);
mManager.setStackFromEnd(true);
mRecycler.setLayoutManager(mManager);
// Set up FirebaseRecyclerAdapter with the Query
Query absencesQuery = getQuery(mDatabase);
mDatabase.addListenerForSingleValueEvent(new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot dataSnapshot) {
// the initial data has been loaded, hide the progress bar
hidefProgressDialog();
}
@Override
public void onCancelled(DatabaseError firebaseError) {
hidefProgressDialog();
}
});
mAdapter = new FirebaseRecyclerAdapter<Attendance, AbsenceViewHolder>(Attendance.class, R.layout.item_absence,
AbsenceViewHolder.class, absencesQuery) {
@Override
protected void populateViewHolder(final AbsenceViewHolder viewHolder, final Attendance model, final int position) {
final DatabaseReference absRef = getRef(position);
// Set click listener for the whole post view
final String absKey = absRef.getKey();
absxy = absRef.getKey();
viewHolder.itemView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Launch PostDetailActivity
Log.d(TAG, "onclick" + " listener");
//Intent intent = new Intent(getActivity(), PostDetailActivity.class);
//intent.putExtra(PostDetailActivity.EXTRA_POST_KEY, absKey);
Toast.makeText(getActivity(), "Onclick " + absKey, Toast.LENGTH_SHORT).show();
//startActivity(intent);
}
});
viewHolder.itemView.setOnLongClickListener(new View.OnLongClickListener() {
@Override
public boolean onLongClick(View v) {
final String datsx = model.getDatsString();
//Log.d(TAG, "datsx " + datsx);
gettimestramp.setValue(ServerValue.TIMESTAMP);
//Log.d(TAG, "ServerValue.TIMESTAMP " + timestampx);
long timestampl = Long.parseLong(timestampx);
long datsl = Long.parseLong(datsx);
long rozdiel = timestampl - datsl;
//Log.d(TAG, "rozdiel " + rozdiel);
Toast.makeText(getActivity(), "Longclick " + absKey,Toast.LENGTH_SHORT).show();
abskeydel = absKey;
if( model.aprv.equals("2")) {
rozdiel=1;
}
if( rozdiel < 180000 ) {
getDialog(abskeydel);
}else{
Toast.makeText(getActivity(), getResources().getString(R.string.cantdel),Toast.LENGTH_SHORT).show();
}
return true;
}
});
// Bind Abstype to ViewHolder
viewHolder.bindToAbsence(model, new View.OnClickListener() {
@Override
public void onClick(View starView) {
// Need to write to both places the post is stored
DatabaseReference globalPostRef = mDatabase.child("posts").child(absRef.getKey());
// Run two transactions
onStarClicked(globalPostRef);
}
});
}
};
mRecycler.setAdapter(mAdapter);
}
// [START post_stars_transaction]
private void onStarClicked(DatabaseReference postRef) {
postRef.runTransaction(new Transaction.Handler() {
@Override
public Transaction.Result doTransaction(MutableData mutableData) {
Post p = mutableData.getValue(Post.class);
if (p == null) {
return Transaction.success(mutableData);
}
if (p.stars.containsKey(getUid())) {
// Unstar the post and remove self from stars
p.starCount = p.starCount - 1;
p.stars.remove(getUid());
} else {
// Star the post and add self to stars
p.starCount = p.starCount + 1;
p.stars.put(getUid(), true);
}
// Set value and report transaction success
mutableData.setValue(p);
return Transaction.success(mutableData);
}
@Override
public void onComplete(DatabaseError databaseError, boolean b,
DataSnapshot dataSnapshot) {
// Transaction completed
Log.d(TAG, "postTransaction:onComplete:" + databaseError);
}
});
}
// [END post_stars_transaction]
@Override
public void onDestroy() {
super.onDestroy();
if (mAdapter != null) {
mAdapter.cleanup();
}
}
public String getUid() {
return FirebaseAuth.getInstance().getCurrentUser().getUid();
}
public abstract Query getQuery(DatabaseReference databaseReference);
// [START deletefan_out]
private void deletePost(String postkey) {
// delete post key from /posts and user-posts/$userid simultaneously
String userId = getUid();
String key = postkey;
String usicox = SettingsActivity.getUsIco(getActivity());
Map<String, Object> childUpdates = new HashMap<>();
childUpdates.put("/absences/" + key, null);
childUpdates.put("/user-absences/" + userId + "/" + key, null);
childUpdates.put("/company-absences/" + usicox + "/" + key, null);
mDatabase.updateChildren(childUpdates);
String keydel = mDatabase.child("deleted-absences").push().getKey();
DeletedAbs deletedabs = new DeletedAbs(usicox, userId, postkey );
Log.d(TAG, "postkey " + postkey);
Map<String, Object> delValues = deletedabs.toMap();
Map<String, Object> childDelUpdates = new HashMap<>();
childDelUpdates.put("/deleted-absences/" + keydel, delValues);
mDatabase.updateChildren(childDelUpdates);
}
// [END delete_fan_out]
private void getDialog(String postkey) {
// custom dialog
final Dialog dialog = new Dialog(getActivity());
dialog.setContentView(R.layout.custom_dialog);
dialog.setTitle(R.string.item);
// set the custom dialog components - text, image and button
String textx = getString(R.string.item) + " " + abskeydel;
TextView text = (TextView) dialog.findViewById(R.id.text);
text.setText(textx);
ImageView image = (ImageView) dialog.findViewById(R.id.image);
image.setImageResource(R.drawable.ic_image_edit);
Button buttonDelete = (Button) dialog.findViewById(R.id.buttonDelete);
// if button is clicked, close the custom dialog
buttonDelete.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
dialog.dismiss();
deletePost(abskeydel);
}
});
Button buttonEdit = (Button) dialog.findViewById(R.id.buttonEdit);
// if button is clicked, close the custom dialog
buttonEdit.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
dialog.dismiss();
Toast.makeText(getActivity(), getResources().getString(R.string.cantedititem), Toast.LENGTH_SHORT).show();
//Intent i = new Intent(getActivity(), NewPostActivity.class);
//Bundle extras = new Bundle();
//extras.putString("editx", "1");
//extras.putString("keyx", abskeydel);
//i.putExtras(extras);
//startActivity(i);
}
});
dialog.show();
}
public void showfProgressDialog() {
if (fProgressDialog == null) {
fProgressDialog = new ProgressDialog(getActivity());
fProgressDialog.setCancelable(isCancelable);
fProgressDialog.setMessage("Loading...");
}
fProgressDialog.show();
}
public void hidefProgressDialog() {
if (fProgressDialog != null && fProgressDialog.isShowing()) {
fProgressDialog.dismiss();
}
}
@Override
public void onResume() {
super.onResume();
isrunning=true;
}
@Override
public void onPause() {
super.onPause();
isrunning=false;
}
}
| {'content_hash': '2de7b6c26d4a4ed64a30d8cb3eec65cb', 'timestamp': '', 'source': 'github', 'line_count': 378, 'max_line_length': 144, 'avg_line_length': 35.71957671957672, 'alnum_prop': 0.5999851873796475, 'repo_name': 'eurosecom/Attendance', 'id': '07ed19b59a88437f88bb72712341ed8a6630d8f8', 'size': '13502', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'app/src/main/java/com/eusecom/attendance/fragment/AbsenceListFragment.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Java', 'bytes': '1190010'}]} |
from __future__ import unicode_literals
from django.conf import settings
from django.db import migrations, models
import django.db.models.deletion
class Migration(migrations.Migration):
initial = True
dependencies = [
('auth', '0007_alter_validators_add_error_messages'),
]
operations = [
migrations.CreateModel(
name='UserProfile',
fields=[
('user', models.OneToOneField(on_delete=django.db.models.deletion.CASCADE, primary_key=True, related_name='profile', serialize=False, to=settings.AUTH_USER_MODEL)),
('camera_type', models.CharField(blank=True, max_length=30)),
('address', models.CharField(blank=True, max_length=60)),
('web_link', models.CharField(blank=True, max_length=70)),
('photo_type', models.CharField(blank=True, max_length=30)),
('social_media', models.CharField(blank=True, max_length=30)),
('region', models.CharField(choices=[('North America', 'North America'), ('Asia', 'Asia'), ('Africa', 'Africa'), ('South America', 'South America'), ('Europe', 'Europe')], default='North America', max_length=30)),
('friends', models.ManyToManyField(related_name='friend_of', to='imager_profile.UserProfile')),
],
),
]
| {'content_hash': 'e1530fb22e9ddd0e6f4714806ab49c7f', 'timestamp': '', 'source': 'github', 'line_count': 30, 'max_line_length': 229, 'avg_line_length': 44.733333333333334, 'alnum_prop': 0.6162444113263785, 'repo_name': 'nadiabahrami/django-imager', 'id': '230ba4c7e8e40918c411c9581d92fc9ada6243bd', 'size': '1414', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'imagersite/imager_profile/migrations/0001_initial.py', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '68384'}, {'name': 'HTML', 'bytes': '27306'}, {'name': 'JavaScript', 'bytes': '14517'}, {'name': 'Python', 'bytes': '37251'}]} |
from ...ags._geoprocessing import *
from ..._abstract import abstract
########################################################################
class analysis(abstract.BaseAGOLClass):
"""
ArcGIS Online is a collaborative, cloud-based platform that lets
members of an organization create, share, and access maps,
applications, and data, including authoritative basemaps published
by Esri. Through ArcGIS Online, you get access to Esri's secure
cloud, where you can manage, create, store, and access hosted web
services.
ArcGIS Online includes the Spatial Analysis service. The Spatial
Analysis service contains a number of tasks, listed below, that you
can access and use in your applications. Using Spatial Analysis
service tasks consumes credits. For more information on credits, see
see Service credits overview which includes access to an interactive
Service Credits Estimator.
Site Reference: https://developers.arcgis.com/rest/analysis/
Inputs:
securityHandler - ArcGIS Online security handler object
url - optional url to the site.
ex: http://www.arcgis.com/sharing/rest
proxy_url - optional proxy IP
proxy_port - optional proxy port required if proxy_url specified
Basic Usage:
import arcrest
import arcrest.agol as agol
if __name__ == "__main__":
username = "username"
password = "password"
sh = arcrest.AGOLTokenSecurityHandler(username, password)
a = agol.analysis(securityHandler=sh)
for task in a.tasks:
if task.name.lower() == "aggregatepoints":
for params in task.parameters:
print params
"""
_proxy_url = None
_proxy_port = None
_url = None
_analysis_url = None
_securityHandler = None
_gpService = None
#----------------------------------------------------------------------
def __init__(self,
securityHandler,
url=None,
proxy_url=None,
proxy_port=None):
"""Constructor"""
if url is None:
self._url = "https://www.arcgis.com/sharing/rest"
else:
if url.find("/sharing/rest") == -1:
url = url + "/sharing/rest"
self._url = url
self._securityHandler = securityHandler
self._proxy_url = proxy_url
self._proxy_port = proxy_port
self.__init_url()
#----------------------------------------------------------------------
def __init_url(self):
"""loads the information into the class"""
portals_self_url = "{}/portals/self".format(self._url)
params = {
"f" :"json"
}
res = self._do_get(url=portals_self_url,
param_dict=params,
securityHandler=self._securityHandler,
proxy_url=self._proxy_url,
proxy_port=self._proxy_port)
if "helperServices" in res:
helper_services = res.get("helperServices")
if "analysis" in helper_services:
analysis_service = helper_services.get("analysis")
if "url" in analysis_service:
self._analysis_url = analysis_service.get("url")
self._gpService = GPService(url=self._analysis_url,
securityHandler=self._securityHandler,
proxy_url=self._proxy_url,
proxy_port=self._proxy_port,
initialize=False)
#----------------------------------------------------------------------
@property
def gpService(self):
"""returns the geoprocessing object"""
if self._gpService is None:
self.__init_url()
return self._gpService
#----------------------------------------------------------------------
@property
def tasks(self):
"""returns the available analysis tasks"""
return self.gpService.tasks
| {'content_hash': 'ae0b663bff1c548f7e3c75fc8e851b0a', 'timestamp': '', 'source': 'github', 'line_count': 99, 'max_line_length': 75, 'avg_line_length': 41.81818181818182, 'alnum_prop': 0.5246376811594203, 'repo_name': 'jgravois/ArcREST', 'id': '4d734fec16834bd31f1e72edebedceac08bc00d8', 'size': '4140', 'binary': False, 'copies': '2', 'ref': 'refs/heads/master', 'path': 'src/arcrest/agol/helperservices/analysis.py', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Python', 'bytes': '1591951'}]} |
package com.loadburn.heron.storage.cache;
import com.google.common.collect.Lists;
import com.loadburn.heron.storage.config.EntityMetadata;
import com.loadburn.heron.utils.StringUtils;
import net.sf.ehcache.search.Query;
import net.sf.ehcache.search.Result;
import java.util.Collection;
import java.util.List;
import java.util.Map;
/**
* @author slacrey ([email protected])
* Date: 13-12-14
*/
public class CacheUtils {
private final static Object cacheLock = new Object();
private final static String REPLACE_CHAR = "_";
private final static String CACHE_NAME_SPLIT_CHAR = ".";
private final static String ARGUMENT_SPLIT_CHAR = "=";
private final static String ARGUMENTS_SPLIT_CHAR = ",";
/**
* 生成缓存Key
*
* @param cacheName 实体类@Cache配置的缓存名称
* @param statement 查询实体类的查询语句
* @param arguments 查询语句的参数
* @return 返回缓存Key字符串
*/
public static String getCacheKey(String cacheName, String statement, Map<String, Object> arguments) {
StringBuilder keyBuilder = new StringBuilder();
String key = StringUtils.replaceAs(statement, REPLACE_CHAR);
keyBuilder.append(cacheName).append(CACHE_NAME_SPLIT_CHAR).append(key);
if (arguments != null) {
keyBuilder.append(CACHE_NAME_SPLIT_CHAR);
for (Map.Entry<String, Object> entry : arguments.entrySet()) {
keyBuilder.append(entry.getKey()).append(ARGUMENT_SPLIT_CHAR).append(entry.getValue()).append(ARGUMENTS_SPLIT_CHAR);
}
}
return keyBuilder.toString();
}
public static void removeCacheKeyLike(ICache cache, String cacheName) {
List<String> keyList = cache.get(EntityMetadata.STORAGE_CACHE_NAME, cacheName);
if (keyList != null) {
cache.removeCollection(EntityMetadata.STORAGE_CACHE_NAME, keyList);
keyList.clear();
}
}
/**
* 获取缓存对象
*
* @param cache 缓存对象
* @return 返回指定对象
*/
public static Object getCacheResult(ICache cache, String key) {
return cache.get(EntityMetadata.STORAGE_CACHE_NAME, key);
}
public static void putCacheResult(ICache cache, String key, Object value) {
cache.put(EntityMetadata.STORAGE_CACHE_NAME, key, value);
}
public static void putCacheStatement(ICache cache, String cacheName, String key) {
synchronized(cacheLock){
List<String> cacheKeyList = cache.get(EntityMetadata.STORAGE_CACHE_NAME, cacheName);
if (cacheKeyList == null) {
cacheKeyList = Lists.newArrayList();
cacheKeyList.add(key);
} else {
if (!cacheKeyList.contains(key)) {
cacheKeyList.add(key);
}
}
cache.put(EntityMetadata.STORAGE_CACHE_NAME, cacheName, cacheKeyList);
}
}
}
| {'content_hash': 'a4f645629b7cc73323332f4c77f2d15b', 'timestamp': '', 'source': 'github', 'line_count': 89, 'max_line_length': 132, 'avg_line_length': 32.348314606741575, 'alnum_prop': 0.6422368878082667, 'repo_name': 'slacrey/heron-project', 'id': 'ee8be98013cb37bd8d2c268c4e3a993a5ad59b42', 'size': '2987', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'heron-storage/src/main/java/com/loadburn/heron/storage/cache/CacheUtils.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'HTML', 'bytes': '1605'}, {'name': 'Java', 'bytes': '376944'}]} |
<?xml version="1.0" encoding="UTF-8" standalone="no"?>
<servico xmlns="http://servicos.gov.br/v3/schema" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://servicos.gov.br/v3/schema ../servico.xsd">
<nome>Alteração Cadastral no CPF</nome>
<sigla/>
<nomes-populares/>
<descricao>Realizar pedido de alteração de dados no CPF em uma conveniada (Banco do Brasil, Caixa Econômica Federal, Correios) ou alteração de endereço em entidades que prestam o serviço gratuitamente.</descricao>
<gratuito/>
<solicitantes/>
<tempo-total-estimado>
<descricao/>
</tempo-total-estimado>
<etapas>
<etapa>
<titulo/>
<descricao/>
<documentos>
<default/>
</documentos>
<custos>
<default/>
</custos>
<canais-de-prestacao>
<default>
<canal-de-prestacao tipo="web">
<descricao>http://www.receita.fazenda.gov.br/PessoaFisica/CPF/CPFEntPublicConven.htm</descricao>
</canal-de-prestacao>
<canal-de-prestacao tipo="presencial">
<descricao>Unidades de Atendimento Conveniadas: Agências da CEF, BB e Correios</descricao>
</canal-de-prestacao>
<canal-de-prestacao tipo="web">
<descricao>[Informações sobre alteração cadastral do CPF](http://www.receita.fazenda.gov.br/PessoaFisica/CPF/CPFAlteracaoDadosCad.htm)</descricao>
</canal-de-prestacao>
</default>
</canais-de-prestacao>
</etapa>
</etapas>
<orgao id="http://estruturaorganizacional.dados.gov.br/id/unidade-organizacional/77"/>
<segmentos-da-sociedade>
<item>Cidadãos</item>
</segmentos-da-sociedade>
<areas-de-interesse>
<item>Economia e Finanças</item>
</areas-de-interesse>
<palavras-chave/>
<legislacoes/>
</servico>
| {'content_hash': '290b7b93aca9aee1ac1ed9b012e8f9cf', 'timestamp': '', 'source': 'github', 'line_count': 46, 'max_line_length': 217, 'avg_line_length': 44.26086956521739, 'alnum_prop': 0.5888998035363457, 'repo_name': 'servicosgoval/cartas-de-servico', 'id': 'e0fa30b85d010994577aa407ff2d82a3993b3ddb', 'size': '2052', 'binary': False, 'copies': '2', 'ref': 'refs/heads/master', 'path': 'cartas-servico/v3/servicos/alteracao-cadastral-no-cpf.xml', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Shell', 'bytes': '846'}]} |
function setEigenvalues( obj, gamma, E )
% SETEIGENVALUES Save eigenvalues of an nlsaKoopmanOperator_diff object
%
% Modified 2020/04/12
% Set eigenvalues using the parent method
setEigenvalues@nlsaKoopmanOperator( obj, gamma )
if nargin == 2 || isempty( E )
return
end
% Set Dirichlet energies
if ~isrow( E ) || ~isnumeric( E )
error( 'Dirichlet energies must be specified as a numeric row vector' )
end
if numel( E ) ~= getNEigenfunction( obj )
msgStr = [ 'Number of Dirichlet energies must be equal to the ' ...
'number of eigenfunctions.' ];
error( msgStr )
end
save( fullfile( getEigenfunctionPath( obj ), getEigenvalueFile( obj ) ), ...
'E', '-append' )
| {'content_hash': '79b5cdec947abff6d69f7ae95d8281c6', 'timestamp': '', 'source': 'github', 'line_count': 23, 'max_line_length': 76, 'avg_line_length': 30.565217391304348, 'alnum_prop': 0.6827880512091038, 'repo_name': 'dg227/NLSA', 'id': 'dc4ec1235a17fbb22a7d3c5c97d3dd844d63dfdd', 'size': '703', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'nlsa/classes/@nlsaKoopmanOperator_diff/setEigenvalues.m', 'mode': '33188', 'license': 'bsd-3-clause', 'language': [{'name': 'Jupyter Notebook', 'bytes': '3276224'}, {'name': 'MATLAB', 'bytes': '1847822'}, {'name': 'Python', 'bytes': '39015'}, {'name': 'Vim Script', 'bytes': '34'}]} |
using System;
using System.Collections.Generic;
using System.Diagnostics.CodeAnalysis;
using System.IO;
using System.Reflection;
using System.Runtime.CompilerServices;
using System.Runtime.InteropServices;
using Godot.NativeInterop;
namespace Godot
{
internal static class DelegateUtils
{
[UnmanagedCallersOnly]
internal static godot_bool DelegateEquals(IntPtr delegateGCHandleA, IntPtr delegateGCHandleB)
{
try
{
var @delegateA = (Delegate?)GCHandle.FromIntPtr(delegateGCHandleA).Target;
var @delegateB = (Delegate?)GCHandle.FromIntPtr(delegateGCHandleB).Target;
return (@delegateA! == @delegateB!).ToGodotBool();
}
catch (Exception e)
{
ExceptionUtils.LogException(e);
return godot_bool.False;
}
}
[UnmanagedCallersOnly]
internal static unsafe void InvokeWithVariantArgs(IntPtr delegateGCHandle, void* trampoline,
godot_variant** args, int argc, godot_variant* outRet)
{
try
{
if (trampoline == null)
{
throw new ArgumentNullException(nameof(trampoline),
"Cannot dynamically invoke delegate because the trampoline is null.");
}
var @delegate = (Delegate)GCHandle.FromIntPtr(delegateGCHandle).Target!;
var trampolineFn = (delegate* managed<object, NativeVariantPtrArgs, out godot_variant, void>)trampoline;
trampolineFn(@delegate, new NativeVariantPtrArgs(args, argc), out godot_variant ret);
*outRet = ret;
}
catch (Exception e)
{
ExceptionUtils.LogException(e);
*outRet = default;
}
}
// TODO: Check if we should be using BindingFlags.DeclaredOnly (would give better reflection performance).
private enum TargetKind : uint
{
Static,
GodotObject,
CompilerGenerated
}
internal static bool TrySerializeDelegate(Delegate @delegate, Collections.Array serializedData)
{
if (@delegate is null)
{
return false;
}
if (@delegate is MulticastDelegate multicastDelegate)
{
bool someDelegatesSerialized = false;
Delegate[] invocationList = multicastDelegate.GetInvocationList();
if (invocationList.Length > 1)
{
var multiCastData = new Collections.Array();
foreach (Delegate oneDelegate in invocationList)
someDelegatesSerialized |= TrySerializeDelegate(oneDelegate, multiCastData);
if (!someDelegatesSerialized)
return false;
serializedData.Add(multiCastData);
return true;
}
}
if (TrySerializeSingleDelegate(@delegate, out byte[]? buffer))
{
serializedData.Add((Span<byte>)buffer);
return true;
}
return false;
}
private static bool TrySerializeSingleDelegate(Delegate @delegate, [MaybeNullWhen(false)] out byte[] buffer)
{
buffer = null;
object? target = @delegate.Target;
switch (target)
{
case null:
{
using (var stream = new MemoryStream())
using (var writer = new BinaryWriter(stream))
{
writer.Write((ulong)TargetKind.Static);
SerializeType(writer, @delegate.GetType());
if (!TrySerializeMethodInfo(writer, @delegate.Method))
return false;
buffer = stream.ToArray();
return true;
}
}
// ReSharper disable once RedundantNameQualifier
case Godot.Object godotObject:
{
using (var stream = new MemoryStream())
using (var writer = new BinaryWriter(stream))
{
writer.Write((ulong)TargetKind.GodotObject);
// ReSharper disable once RedundantCast
writer.Write((ulong)godotObject.GetInstanceId());
SerializeType(writer, @delegate.GetType());
if (!TrySerializeMethodInfo(writer, @delegate.Method))
return false;
buffer = stream.ToArray();
return true;
}
}
default:
{
Type targetType = target.GetType();
if (targetType.IsDefined(typeof(CompilerGeneratedAttribute), true))
{
// Compiler generated. Probably a closure. Try to serialize it.
using (var stream = new MemoryStream())
using (var writer = new BinaryWriter(stream))
{
writer.Write((ulong)TargetKind.CompilerGenerated);
SerializeType(writer, targetType);
SerializeType(writer, @delegate.GetType());
if (!TrySerializeMethodInfo(writer, @delegate.Method))
return false;
FieldInfo[] fields = targetType.GetFields(BindingFlags.Instance | BindingFlags.Public);
writer.Write(fields.Length);
foreach (FieldInfo field in fields)
{
Type fieldType = field.GetType();
Variant.Type variantType = GD.TypeToVariantType(fieldType);
if (variantType == Variant.Type.Nil)
return false;
static byte[] VarToBytes(in godot_variant var)
{
NativeFuncs.godotsharp_var_to_bytes(var, false.ToGodotBool(), out var varBytes);
using (varBytes)
return Marshaling.ConvertNativePackedByteArrayToSystemArray(varBytes);
}
writer.Write(field.Name);
var fieldValue = field.GetValue(target);
using var fieldValueVariant = Marshaling.ConvertManagedObjectToVariant(fieldValue);
byte[] valueBuffer = VarToBytes(fieldValueVariant);
writer.Write(valueBuffer.Length);
writer.Write(valueBuffer);
}
buffer = stream.ToArray();
return true;
}
}
return false;
}
}
}
private static bool TrySerializeMethodInfo(BinaryWriter writer, MethodInfo methodInfo)
{
SerializeType(writer, methodInfo.DeclaringType);
writer.Write(methodInfo.Name);
int flags = 0;
if (methodInfo.IsPublic)
flags |= (int)BindingFlags.Public;
else
flags |= (int)BindingFlags.NonPublic;
if (methodInfo.IsStatic)
flags |= (int)BindingFlags.Static;
else
flags |= (int)BindingFlags.Instance;
writer.Write(flags);
Type returnType = methodInfo.ReturnType;
bool hasReturn = methodInfo.ReturnType != typeof(void);
writer.Write(hasReturn);
if (hasReturn)
SerializeType(writer, returnType);
ParameterInfo[] parameters = methodInfo.GetParameters();
writer.Write(parameters.Length);
if (parameters.Length > 0)
{
for (int i = 0; i < parameters.Length; i++)
SerializeType(writer, parameters[i].ParameterType);
}
return true;
}
private static void SerializeType(BinaryWriter writer, Type? type)
{
if (type == null)
{
int genericArgumentsCount = -1;
writer.Write(genericArgumentsCount);
}
else if (type.IsGenericType)
{
Type genericTypeDef = type.GetGenericTypeDefinition();
Type[] genericArgs = type.GetGenericArguments();
int genericArgumentsCount = genericArgs.Length;
writer.Write(genericArgumentsCount);
writer.Write(genericTypeDef.Assembly.GetName().Name ?? "");
writer.Write(genericTypeDef.FullName ?? genericTypeDef.ToString());
for (int i = 0; i < genericArgs.Length; i++)
SerializeType(writer, genericArgs[i]);
}
else
{
int genericArgumentsCount = 0;
writer.Write(genericArgumentsCount);
writer.Write(type.Assembly.GetName().Name ?? "");
writer.Write(type.FullName ?? type.ToString());
}
}
[UnmanagedCallersOnly]
internal static unsafe godot_bool TrySerializeDelegateWithGCHandle(IntPtr delegateGCHandle,
godot_array* nSerializedData)
{
try
{
var serializedData = Collections.Array.CreateTakingOwnershipOfDisposableValue(
NativeFuncs.godotsharp_array_new_copy(*nSerializedData));
var @delegate = (Delegate)GCHandle.FromIntPtr(delegateGCHandle).Target!;
return TrySerializeDelegate(@delegate, serializedData)
.ToGodotBool();
}
catch (Exception e)
{
ExceptionUtils.LogException(e);
return godot_bool.False;
}
}
[UnmanagedCallersOnly]
internal static unsafe godot_bool TryDeserializeDelegateWithGCHandle(godot_array* nSerializedData,
IntPtr* delegateGCHandle)
{
try
{
var serializedData = Collections.Array.CreateTakingOwnershipOfDisposableValue(
NativeFuncs.godotsharp_array_new_copy(*nSerializedData));
if (TryDeserializeDelegate(serializedData, out Delegate? @delegate))
{
*delegateGCHandle = GCHandle.ToIntPtr(CustomGCHandle.AllocStrong(@delegate));
return godot_bool.True;
}
else
{
*delegateGCHandle = IntPtr.Zero;
return godot_bool.False;
}
}
catch (Exception e)
{
ExceptionUtils.LogException(e);
*delegateGCHandle = default;
return godot_bool.False;
}
}
internal static bool TryDeserializeDelegate(Collections.Array serializedData,
[MaybeNullWhen(false)] out Delegate @delegate)
{
@delegate = null;
if (serializedData.Count == 1)
{
var elem = serializedData[0].Obj;
if (elem == null)
return false;
if (elem is Collections.Array multiCastData)
return TryDeserializeDelegate(multiCastData, out @delegate);
return TryDeserializeSingleDelegate((byte[])elem, out @delegate);
}
var delegates = new List<Delegate>(serializedData.Count);
foreach (Variant variantElem in serializedData)
{
var elem = variantElem.Obj;
if (elem == null)
continue;
if (elem is Collections.Array multiCastData)
{
if (TryDeserializeDelegate(multiCastData, out Delegate? oneDelegate))
delegates.Add(oneDelegate);
}
else
{
if (TryDeserializeSingleDelegate((byte[])elem, out Delegate? oneDelegate))
delegates.Add(oneDelegate);
}
}
if (delegates.Count <= 0)
return false;
@delegate = delegates.Count == 1 ? delegates[0] : Delegate.Combine(delegates.ToArray())!;
return true;
}
private static bool TryDeserializeSingleDelegate(byte[] buffer, [MaybeNullWhen(false)] out Delegate @delegate)
{
@delegate = null;
using (var stream = new MemoryStream(buffer, writable: false))
using (var reader = new BinaryReader(stream))
{
var targetKind = (TargetKind)reader.ReadUInt64();
switch (targetKind)
{
case TargetKind.Static:
{
Type? delegateType = DeserializeType(reader);
if (delegateType == null)
return false;
if (!TryDeserializeMethodInfo(reader, out MethodInfo? methodInfo))
return false;
@delegate = Delegate.CreateDelegate(delegateType, null, methodInfo, throwOnBindFailure: false);
if (@delegate == null)
return false;
return true;
}
case TargetKind.GodotObject:
{
ulong objectId = reader.ReadUInt64();
// ReSharper disable once RedundantNameQualifier
Godot.Object godotObject = GD.InstanceFromId(objectId);
if (godotObject == null)
return false;
Type? delegateType = DeserializeType(reader);
if (delegateType == null)
return false;
if (!TryDeserializeMethodInfo(reader, out MethodInfo? methodInfo))
return false;
@delegate = Delegate.CreateDelegate(delegateType, godotObject, methodInfo,
throwOnBindFailure: false);
if (@delegate == null)
return false;
return true;
}
case TargetKind.CompilerGenerated:
{
Type? targetType = DeserializeType(reader);
if (targetType == null)
return false;
Type? delegateType = DeserializeType(reader);
if (delegateType == null)
return false;
if (!TryDeserializeMethodInfo(reader, out MethodInfo? methodInfo))
return false;
int fieldCount = reader.ReadInt32();
object recreatedTarget = Activator.CreateInstance(targetType)!;
for (int i = 0; i < fieldCount; i++)
{
string name = reader.ReadString();
int valueBufferLength = reader.ReadInt32();
byte[] valueBuffer = reader.ReadBytes(valueBufferLength);
FieldInfo? fieldInfo = targetType.GetField(name,
BindingFlags.Instance | BindingFlags.Public);
fieldInfo?.SetValue(recreatedTarget, GD.BytesToVar(valueBuffer));
}
@delegate = Delegate.CreateDelegate(delegateType, recreatedTarget, methodInfo,
throwOnBindFailure: false);
if (@delegate == null)
return false;
return true;
}
default:
return false;
}
}
}
private static bool TryDeserializeMethodInfo(BinaryReader reader,
[MaybeNullWhen(false)] out MethodInfo methodInfo)
{
methodInfo = null;
Type? declaringType = DeserializeType(reader);
if (declaringType == null)
return false;
string methodName = reader.ReadString();
int flags = reader.ReadInt32();
bool hasReturn = reader.ReadBoolean();
Type? returnType = hasReturn ? DeserializeType(reader) : typeof(void);
int parametersCount = reader.ReadInt32();
if (parametersCount > 0)
{
var parameterTypes = new Type[parametersCount];
for (int i = 0; i < parametersCount; i++)
{
Type? parameterType = DeserializeType(reader);
if (parameterType == null)
return false;
parameterTypes[i] = parameterType;
}
methodInfo = declaringType.GetMethod(methodName, (BindingFlags)flags, null, parameterTypes, null);
return methodInfo != null && methodInfo.ReturnType == returnType;
}
methodInfo = declaringType.GetMethod(methodName, (BindingFlags)flags);
return methodInfo != null && methodInfo.ReturnType == returnType;
}
private static Type? DeserializeType(BinaryReader reader)
{
int genericArgumentsCount = reader.ReadInt32();
if (genericArgumentsCount == -1)
return null;
string assemblyName = reader.ReadString();
if (assemblyName.Length == 0)
{
GD.PushError($"Missing assembly name of type when attempting to deserialize delegate");
return null;
}
string typeFullName = reader.ReadString();
var type = ReflectionUtils.FindTypeInLoadedAssemblies(assemblyName, typeFullName);
if (type == null)
return null; // Type not found
if (genericArgumentsCount != 0)
{
var genericArgumentTypes = new Type[genericArgumentsCount];
for (int i = 0; i < genericArgumentsCount; i++)
{
Type? genericArgumentType = DeserializeType(reader);
if (genericArgumentType == null)
return null;
genericArgumentTypes[i] = genericArgumentType;
}
type = type.MakeGenericType(genericArgumentTypes);
}
return type;
}
}
}
| {'content_hash': 'a91f82711e5326e2322b94a3fab9fd5a', 'timestamp': '', 'source': 'github', 'line_count': 539, 'max_line_length': 120, 'avg_line_length': 36.12615955473098, 'alnum_prop': 0.4948644207066557, 'repo_name': 'BastiaanOlij/godot', 'id': 'd19e0c08f20626229583e03764373e4591ebcc81', 'size': '19490', 'binary': False, 'copies': '4', 'ref': 'refs/heads/master', 'path': 'modules/mono/glue/GodotSharp/GodotSharp/Core/DelegateUtils.cs', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'AIDL', 'bytes': '1633'}, {'name': 'C', 'bytes': '1045182'}, {'name': 'C#', 'bytes': '1652166'}, {'name': 'C++', 'bytes': '40241361'}, {'name': 'CMake', 'bytes': '606'}, {'name': 'GAP', 'bytes': '62'}, {'name': 'GDScript', 'bytes': '71651'}, {'name': 'GLSL', 'bytes': '873103'}, {'name': 'Java', 'bytes': '596800'}, {'name': 'JavaScript', 'bytes': '192587'}, {'name': 'Kotlin', 'bytes': '93312'}, {'name': 'Makefile', 'bytes': '1421'}, {'name': 'Objective-C', 'bytes': '20550'}, {'name': 'Objective-C++', 'bytes': '400430'}, {'name': 'PowerShell', 'bytes': '2713'}, {'name': 'Python', 'bytes': '482048'}, {'name': 'Shell', 'bytes': '33724'}]} |
<component name="libraryTable">
<library name="Maven: org.apache.servicemix.bundles:org.apache.servicemix.bundles.ezmorph:1.0.6_1">
<CLASSES>
<root url="jar://$MAVEN_REPOSITORY$/org/apache/servicemix/bundles/org.apache.servicemix.bundles.ezmorph/1.0.6_1/org.apache.servicemix.bundles.ezmorph-1.0.6_1.jar!/" />
</CLASSES>
<JAVADOC>
<root url="jar://$MAVEN_REPOSITORY$/org/apache/servicemix/bundles/org.apache.servicemix.bundles.ezmorph/1.0.6_1/org.apache.servicemix.bundles.ezmorph-1.0.6_1-javadoc.jar!/" />
</JAVADOC>
<SOURCES>
<root url="jar://$MAVEN_REPOSITORY$/org/apache/servicemix/bundles/org.apache.servicemix.bundles.ezmorph/1.0.6_1/org.apache.servicemix.bundles.ezmorph-1.0.6_1-sources.jar!/" />
</SOURCES>
</library>
</component> | {'content_hash': '0a6310001c15b92abc8c19f0ac854c1b', 'timestamp': '', 'source': 'github', 'line_count': 13, 'max_line_length': 181, 'avg_line_length': 60.30769230769231, 'alnum_prop': 0.7142857142857143, 'repo_name': 'hitakaken/bigfoot', 'id': '1baf1da979980c731851187425b777f75a1d0793', 'size': '784', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': '.idea/libraries/Maven__org_apache_servicemix_bundles_org_apache_servicemix_bundles_ezmorph_1_0_6_1.xml', 'mode': '33188', 'license': 'apache-2.0', 'language': []} |
import { Component, OnInit } from '@angular/core';
@Component({
selector: 'app-comp-1831',
templateUrl: './comp-1831.component.html',
styleUrls: ['./comp-1831.component.css']
})
export class Comp1831Component implements OnInit {
constructor() { }
ngOnInit() {
}
}
| {'content_hash': 'f4933cc54ce870303d4d89b0d2f313a4', 'timestamp': '', 'source': 'github', 'line_count': 17, 'max_line_length': 50, 'avg_line_length': 16.58823529411765, 'alnum_prop': 0.6666666666666666, 'repo_name': 'angular/angular-cli-stress-test', 'id': '3711e2a7aebff0ccdefa211d3b85be97223b35de', 'size': '484', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/app/components/comp-1831/comp-1831.component.ts', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '1040888'}, {'name': 'HTML', 'bytes': '300322'}, {'name': 'JavaScript', 'bytes': '2404'}, {'name': 'TypeScript', 'bytes': '8535506'}]} |
<?php
namespace ODesk\HomeTaskBundle\Tests\Services;
use ODesk\HomeTaskBundle\Services\MatrixUtil;
class CalculatorTest extends \PHPUnit_Framework_TestCase
{
public function testGenSpiralMatrix()
{
$word = 'test';
$this->assertEquals(array(
array('t', 'e', 's'),
array('t', 't', 't'),
array('s', 'e', 't')
), MatrixUtil::genSpiralMatrix($word, 3));
$this->assertEquals(array(
array('t', 'e'),
array('t', 's')
), MatrixUtil::genSpiralMatrix($word, 2));
$this->assertEquals(array(array('t')), MatrixUtil::genSpiralMatrix($word, 1));
try {
MatrixUtil::genSpiralMatrix('', null);
} catch (\InvalidArgumentException $expected) {
$this->assertEquals($expected->getMessage(), 'Word can\'t be empty');
} catch (\Exception $e) {
$this->fail('An expected exception has not been raised.');
}
}
} | {'content_hash': '32d883323173cf5b76211e2cf3da247b', 'timestamp': '', 'source': 'github', 'line_count': 33, 'max_line_length': 86, 'avg_line_length': 29.636363636363637, 'alnum_prop': 0.5603271983640081, 'repo_name': 'abdula/symfony-play', 'id': '6b6a6d2197a37472d9a970705d3044f07d89d5ca', 'size': '978', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/ODesk/HomeTaskBundle/Tests/Services/MatrixUtilTest.php', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '564'}, {'name': 'PHP', 'bytes': '48018'}]} |
Deletes the user.
## SYNTAX
### ClientObject (Default)
```
Remove-SPClientUser [-ClientContext <ClientContext>] [-ClientObject] <User>
```
### Identity
```
Remove-SPClientUser [-ClientContext <ClientContext>] -Identity <Int32>
```
### Name
```
Remove-SPClientUser [-ClientContext <ClientContext>] -Name <String>
```
### Email
```
Remove-SPClientUser [-ClientContext <ClientContext>] -Email <String>
```
## DESCRIPTION
The Remove-SPClientUser function removes the user from the site.
If the user could not be found, throws exception.
## EXAMPLES
### -------------------------- Example 1 --------------------------
```
Remove-SPClientUser $user
```
### -------------------------- Example 2 --------------------------
```
Remove-SPClientUser -Identity 7
```
### -------------------------- Example 3 --------------------------
```
Remove-SPClientUser -Name "i:0#.f|membership|[email protected]"
```
### -------------------------- Example 4 --------------------------
```
Remove-SPClientUser -Email "[email protected]"
```
## PARAMETERS
### -ClientContext
Indicates the client context.
If not specified, uses a default context.
```yaml
Type: ClientContext
Parameter Sets: (All)
Aliases:
Required: False
Position: Named
Default value: $SPClient.ClientContext
Accept pipeline input: False
Accept wildcard characters: False
```
### -ClientObject
Indicates the user to delete.
```yaml
Type: User
Parameter Sets: ClientObject
Aliases:
Required: True
Position: 1
Default value: None
Accept pipeline input: True (ByValue)
Accept wildcard characters: False
```
### -Identity
Indicates the user ID.
```yaml
Type: Int32
Parameter Sets: Identity
Aliases: Id
Required: True
Position: Named
Default value: 0
Accept pipeline input: False
Accept wildcard characters: False
```
### -Name
Indicates the user login name.
```yaml
Type: String
Parameter Sets: Name
Aliases: LoginName
Required: True
Position: Named
Default value: None
Accept pipeline input: False
Accept wildcard characters: False
```
### -Email
Indicates the user E-mail.
```yaml
Type: String
Parameter Sets: Email
Aliases:
Required: True
Position: Named
Default value: None
Accept pipeline input: False
Accept wildcard characters: False
```
## INPUTS
### None or Microsoft.SharePoint.Client.User
## OUTPUTS
### None
## NOTES
## RELATED LINKS
[https://github.com/karamem0/SPClient/blob/master/doc/Remove-SPClientUser.md](https://github.com/karamem0/SPClient/blob/master/doc/Remove-SPClientUser.md)
| {'content_hash': 'b74a1003f6240667694b32d6eb0a5ca0', 'timestamp': '', 'source': 'github', 'line_count': 142, 'max_line_length': 154, 'avg_line_length': 17.45774647887324, 'alnum_prop': 0.6696248487293264, 'repo_name': 'karamem0/SPClient', 'id': '332e891ed962f1cdaf24a00cf689f3e6ac4d444c', 'size': '2514', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'doc/Remove-SPClientUser.md', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'C#', 'bytes': '20908'}, {'name': 'PowerShell', 'bytes': '579806'}]} |
#ifndef ScrollingTreeNode_h
#define ScrollingTreeNode_h
#if ENABLE(THREADED_SCROLLING)
#include "IntRect.h"
#include "ScrollTypes.h"
#include "ScrollingCoordinator.h"
#include <wtf/PassOwnPtr.h>
namespace WebCore {
class ScrollingTreeNode {
public:
explicit ScrollingTreeNode(ScrollingTree*);
virtual ~ScrollingTreeNode();
virtual void update(ScrollingStateNode*) = 0;
ScrollingNodeID scrollingNodeID() const { return m_nodeID; }
void setScrollingNodeID(ScrollingNodeID nodeID) { m_nodeID = nodeID; }
ScrollingTreeNode* parent() const { return m_parent; }
void setParent(ScrollingTreeNode* parent) { m_parent = parent; }
void appendChild(PassOwnPtr<ScrollingTreeNode>);
void removeChild(ScrollingTreeNode*);
protected:
ScrollingTree* scrollingTree() const { return m_scrollingTree; }
private:
ScrollingTree* m_scrollingTree;
ScrollingNodeID m_nodeID;
ScrollingTreeNode* m_parent;
OwnPtr<Vector<OwnPtr<ScrollingTreeNode> > > m_children;
};
} // namespace WebCore
#endif // ENABLE(THREADED_SCROLLING)
#endif // ScrollingTreeNode_h
| {'content_hash': '82731aa9bcaba3cf1ee315825b4f04a0', 'timestamp': '', 'source': 'github', 'line_count': 47, 'max_line_length': 74, 'avg_line_length': 23.4468085106383, 'alnum_prop': 0.7413793103448276, 'repo_name': 'yoavweiss/RespImg-WebCore', 'id': '46addc40eb1c44ab456fa5f334cd410b08b49684', 'size': '2456', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'page/scrolling/ScrollingTreeNode.h', 'mode': '33188', 'license': 'bsd-2-clause', 'language': [{'name': 'Assembly', 'bytes': '1301'}, {'name': 'C', 'bytes': '2369715'}, {'name': 'C++', 'bytes': '39064862'}, {'name': 'JavaScript', 'bytes': '3763760'}, {'name': 'Objective-C', 'bytes': '2038598'}, {'name': 'Perl', 'bytes': '768866'}, {'name': 'Prolog', 'bytes': '519'}, {'name': 'Python', 'bytes': '210630'}, {'name': 'Ruby', 'bytes': '1927'}, {'name': 'Shell', 'bytes': '8214'}]} |
jQuery(document).ready(function() {
jQuery(function(){
//Registration form validation
jQuery('#registration_form').validate();
});
}); | {'content_hash': 'ae5e8d5c5feedc9277f6e5c7d17c0087', 'timestamp': '', 'source': 'github', 'line_count': 6, 'max_line_length': 42, 'avg_line_length': 23.5, 'alnum_prop': 0.6879432624113475, 'repo_name': 'yoanngern/iahm_2016', 'id': 'dfc6d27a303a55e519c0a06cb8bff365f8718620', 'size': '142', 'binary': False, 'copies': '8', 'ref': 'refs/heads/master', 'path': 'wp-content/plugins/event-espresso-core-reg/core/templates/global_assets/scripts/validation.js', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'ApacheConf', 'bytes': '14'}, {'name': 'CSS', 'bytes': '2370838'}, {'name': 'HTML', 'bytes': '1771'}, {'name': 'JavaScript', 'bytes': '3225493'}, {'name': 'PHP', 'bytes': '21389164'}]} |
"""
raven.contrib.django.handlers
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
:copyright: (c) 2010 by the Sentry Team, see AUTHORS for more details.
:license: BSD, see LICENSE for more details.
"""
from __future__ import absolute_import
import logging
from raven.handlers.logging import SentryHandler as BaseSentryHandler
class SentryHandler(BaseSentryHandler):
def __init__(self):
logging.Handler.__init__(self)
def _get_client(self):
from raven.contrib.django.models import client
return client
client = property(_get_client)
def _emit(self, record):
from raven.contrib.django.middleware import SentryLogMiddleware
# Fetch the request from a threadlocal variable, if available
request = getattr(record, 'request', getattr(SentryLogMiddleware.thread, 'request', None))
return super(SentryHandler, self)._emit(record, request=request)
| {'content_hash': '419af02943a9850ba3ac01aa19fcdddc', 'timestamp': '', 'source': 'github', 'line_count': 32, 'max_line_length': 98, 'avg_line_length': 28.25, 'alnum_prop': 0.6891592920353983, 'repo_name': 'alex/raven', 'id': '275aa2769d16647d3716eb91043949de447e937c', 'size': '904', 'binary': False, 'copies': '3', 'ref': 'refs/heads/master', 'path': 'raven/contrib/django/handlers.py', 'mode': '33188', 'license': 'bsd-3-clause', 'language': [{'name': 'Python', 'bytes': '216588'}]} |
require 'unit_spec_helper'
describe Rpush::Client::ActiveRecord::Apns::App do
it_behaves_like 'Rpush::Client::Apns::App'
it_behaves_like 'Rpush::Client::ActiveRecord::App'
end if active_record?
| {'content_hash': '8ba8c714ccfd3447ed0d9d4b843e0294', 'timestamp': '', 'source': 'github', 'line_count': 6, 'max_line_length': 52, 'avg_line_length': 33.166666666666664, 'alnum_prop': 0.7437185929648241, 'repo_name': 'rpush/rpush', 'id': '97409056d2f47682b9dabdc252ce8d43e72633d1', 'size': '199', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'spec/unit/client/active_record/apns/app_spec.rb', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Ruby', 'bytes': '458658'}]} |
import React, { Component } from 'react';
import PropTypes from 'prop-types';
import classNames from 'classnames';
import '../../assets/scss/SimpleBanner.css';
class SimpleBannerComponent extends Component {
static propTypes = {
classNameBanner: PropTypes.oneOfType([PropTypes.object, PropTypes.string]),
classNameBannerTitle: PropTypes.oneOfType([PropTypes.object, PropTypes.string]),
classNameBannerTitleUpperText: PropTypes.oneOfType([PropTypes.object, PropTypes.string]),
classNameBannerTitleLowerText: PropTypes.oneOfType([PropTypes.object, PropTypes.string]),
upperText: PropTypes.string,
lowerText: PropTypes.string,
};
static defaultProps = {
classNameBanner: '',
classNameBannerTitle: '',
classNameBannerTitleUpperText: '',
classNameBannerTitleLowerText: '',
upperText: '',
lowerText: '',
};
render() {
const {
classNameBanner,
classNameBannerTitle,
classNameBannerTitleUpperText,
classNameBannerTitleLowerText,
upperText,
lowerText,
} = this.props;
const cls = {
banner: classNames('simple-banner', classNameBanner),
bannerOverlay: 'simple-banner__overlay',
bannerTitle: classNames('simple-banner__title', classNameBannerTitle),
bannerTitleTextLight: classNames('simple-banner__title__text simple-banner__title__text--light animated fadeInUp', classNameBannerTitleUpperText),
bannerSmallTitleText: classNames('simple-banner__title__text simple-banner__title__text--small animated fadeInDown', classNameBannerTitleLowerText),
};
return (
<section className={cls.banner} id={'banner'}>
<div className={cls.bannerOverlay} />
<div className={cls.bannerTitle}>
<span className={cls.bannerSmallTitleText}>{upperText}</span>
<span className={cls.bannerTitleTextLight}>{lowerText}</span>
</div>
</section>
)
}
}
export default SimpleBannerComponent;
| {'content_hash': '5577a4c05ad9e7263c9158b489acb62e', 'timestamp': '', 'source': 'github', 'line_count': 57, 'max_line_length': 154, 'avg_line_length': 34.49122807017544, 'alnum_prop': 0.7070193285859614, 'repo_name': 'fcgomes92/pousadacaminhodosventos', 'id': 'dd467b02752a16d8ebc2a46f579ce1289cde1930', 'size': '1966', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/Components/SimpleBanner/SimpleBannerComponent.js', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '33280'}, {'name': 'HTML', 'bytes': '193414'}, {'name': 'JavaScript', 'bytes': '72032'}]} |
<?xml version="1.0" encoding="UTF-8"?>
<project version="4">
<component name="JavadocGenerationManager">
<option name="OUTPUT_DIRECTORY" value="$USER_HOME$/Desktop" />
<option name="OPTION_SCOPE" value="protected" />
<option name="OPTION_HIERARCHY" value="true" />
<option name="OPTION_NAVIGATOR" value="true" />
<option name="OPTION_INDEX" value="true" />
<option name="OPTION_SEPARATE_INDEX" value="true" />
<option name="OPTION_DOCUMENT_TAG_USE" value="true" />
<option name="OPTION_DOCUMENT_TAG_AUTHOR" value="true" />
<option name="OPTION_DOCUMENT_TAG_VERSION" value="true" />
<option name="OPTION_DOCUMENT_TAG_DEPRECATED" value="true" />
<option name="OPTION_DEPRECATED_LIST" value="true" />
<option name="OTHER_OPTIONS" />
<option name="HEAP_SIZE" />
<option name="LOCALE" />
<option name="OPEN_IN_BROWSER" value="true" />
<option name="OPTION_INCLUDE_LIBS" value="false" />
</component>
<component name="ProjectKey">
<option name="state" value="project://e79810c8-c5c8-43b1-b19c-90c1f4095425" />
</component>
<component name="ProjectRootManager" version="2" languageLevel="JDK_1_8" project-jdk-name="1.8" project-jdk-type="JavaSDK">
<output url="file://$PROJECT_DIR$/out" />
</component>
</project> | {'content_hash': 'e55c343b778e2d820dce6b35d5e54d9e', 'timestamp': '', 'source': 'github', 'line_count': 27, 'max_line_length': 125, 'avg_line_length': 47.77777777777778, 'alnum_prop': 0.6682170542635659, 'repo_name': 'sisgandarli/N-Queens', 'id': 'b3f9c1f58b091047e5504742b7d57f62dd06b7bd', 'size': '1290', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': '.idea/misc.xml', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'HTML', 'bytes': '188'}, {'name': 'Java', 'bytes': '10500'}]} |
namespace sync_file_system {
namespace drive {
namespace {
const base::FilePath::CharType kV0FormatPathPrefix[] =
FILE_PATH_LITERAL("drive/");
const char kWapiFileIdPrefix[] = "file:";
const char kWapiFolderIdPrefix[] = "folder:";
std::string RemovePrefix(const std::string& str, const std::string& prefix) {
if (StartsWithASCII(str, prefix, true))
return std::string(str.begin() + prefix.size(), str.end());
return str;
}
} // namespace
bool ParseV0FormatFileSystemURL(const GURL& url,
GURL* origin,
base::FilePath* path) {
fileapi::FileSystemType mount_type;
base::FilePath virtual_path;
if (!fileapi::FileSystemURL::ParseFileSystemSchemeURL(
url, origin, &mount_type, &virtual_path) ||
mount_type != fileapi::kFileSystemTypeExternal) {
NOTREACHED() << "Failed to parse filesystem scheme URL " << url.spec();
return false;
}
base::FilePath::StringType prefix =
base::FilePath(kV0FormatPathPrefix).NormalizePathSeparators().value();
if (virtual_path.value().substr(0, prefix.size()) != prefix)
return false;
*path = base::FilePath(virtual_path.value().substr(prefix.size()));
return true;
}
std::string AddWapiFilePrefix(const std::string& resource_id) {
DCHECK(!StartsWithASCII(resource_id, kWapiFileIdPrefix, true));
DCHECK(!StartsWithASCII(resource_id, kWapiFolderIdPrefix, true));
if (resource_id.empty() ||
StartsWithASCII(resource_id, kWapiFileIdPrefix, true) ||
StartsWithASCII(resource_id, kWapiFolderIdPrefix, true))
return resource_id;
return kWapiFileIdPrefix + resource_id;
}
std::string AddWapiFolderPrefix(const std::string& resource_id) {
DCHECK(!StartsWithASCII(resource_id, kWapiFileIdPrefix, true));
DCHECK(!StartsWithASCII(resource_id, kWapiFolderIdPrefix, true));
if (resource_id.empty() ||
StartsWithASCII(resource_id, kWapiFileIdPrefix, true) ||
StartsWithASCII(resource_id, kWapiFolderIdPrefix, true))
return resource_id;
return kWapiFolderIdPrefix + resource_id;
}
std::string AddWapiIdPrefix(const std::string& resource_id,
DriveMetadata_ResourceType type) {
switch (type) {
case DriveMetadata_ResourceType_RESOURCE_TYPE_FILE:
return AddWapiFilePrefix(resource_id);
case DriveMetadata_ResourceType_RESOURCE_TYPE_FOLDER:
return AddWapiFolderPrefix(resource_id);
}
NOTREACHED();
return resource_id;
}
std::string RemoveWapiIdPrefix(const std::string& resource_id) {
if (StartsWithASCII(resource_id, kWapiFileIdPrefix, true))
return RemovePrefix(resource_id, kWapiFileIdPrefix);
if (StartsWithASCII(resource_id, kWapiFolderIdPrefix, true))
return RemovePrefix(resource_id, kWapiFolderIdPrefix);
return resource_id;
}
SyncStatusCode MigrateDatabaseFromV0ToV1(leveldb::DB* db) {
// Version 0 database format:
// key: "CHANGE_STAMP"
// value: <Largest Changestamp>
//
// key: "SYNC_ROOT_DIR"
// value: <Resource ID of the sync root directory>
//
// key: "METADATA: " +
// <FileSystemURL serialized by SerializeSyncableFileSystemURL>
// value: <Serialized DriveMetadata>
//
// key: "BSYNC_ORIGIN: " + <URL string of a batch sync origin>
// value: <Resource ID of the drive directory for the origin>
//
// key: "ISYNC_ORIGIN: " + <URL string of a incremental sync origin>
// value: <Resource ID of the drive directory for the origin>
//
// Version 1 database format (changed keys/fields are marked with '*'):
// * key: "VERSION" (new)
// * value: 1
//
// key: "CHANGE_STAMP"
// value: <Largest Changestamp>
//
// key: "SYNC_ROOT_DIR"
// value: <Resource ID of the sync root directory>
//
// * key: "METADATA: " + <Origin and URL> (changed)
// * value: <Serialized DriveMetadata>
//
// key: "BSYNC_ORIGIN: " + <URL string of a batch sync origin>
// value: <Resource ID of the drive directory for the origin>
//
// key: "ISYNC_ORIGIN: " + <URL string of a incremental sync origin>
// value: <Resource ID of the drive directory for the origin>
//
// key: "DISABLED_ORIGIN: " + <URL string of a disabled origin>
// value: <Resource ID of the drive directory for the origin>
const char kDatabaseVersionKey[] = "VERSION";
const char kDriveMetadataKeyPrefix[] = "METADATA: ";
const char kMetadataKeySeparator = ' ';
leveldb::WriteBatch write_batch;
write_batch.Put(kDatabaseVersionKey, "1");
scoped_ptr<leveldb::Iterator> itr(db->NewIterator(leveldb::ReadOptions()));
for (itr->Seek(kDriveMetadataKeyPrefix); itr->Valid(); itr->Next()) {
std::string key = itr->key().ToString();
if (!StartsWithASCII(key, kDriveMetadataKeyPrefix, true))
break;
std::string serialized_url(RemovePrefix(key, kDriveMetadataKeyPrefix));
GURL origin;
base::FilePath path;
bool success = ParseV0FormatFileSystemURL(
GURL(serialized_url), &origin, &path);
DCHECK(success) << serialized_url;
std::string new_key = kDriveMetadataKeyPrefix + origin.spec() +
kMetadataKeySeparator + path.AsUTF8Unsafe();
write_batch.Put(new_key, itr->value());
write_batch.Delete(key);
}
return LevelDBStatusToSyncStatusCode(
db->Write(leveldb::WriteOptions(), &write_batch));
}
SyncStatusCode MigrateDatabaseFromV1ToV2(leveldb::DB* db) {
// Strips prefix of WAPI resource ID, and discards batch sync origins.
// (i.e. "file:xxxx" => "xxxx", "folder:yyyy" => "yyyy")
//
// Version 2 database format (changed keys/fields are marked with '*'):
// key: "VERSION"
// * value: 2
//
// key: "CHANGE_STAMP"
// value: <Largest Changestamp>
//
// key: "SYNC_ROOT_DIR"
// * value: <Resource ID of the sync root directory> (striped)
//
// key: "METADATA: " + <Origin and URL>
// * value: <Serialized DriveMetadata> (stripped)
//
// * key: "BSYNC_ORIGIN: " + <URL string of a batch sync origin> (deleted)
// * value: <Resource ID of the drive directory for the origin> (deleted)
//
// key: "ISYNC_ORIGIN: " + <URL string of a incremental sync origin>
// * value: <Resource ID of the drive directory for the origin> (stripped)
//
// key: "DISABLED_ORIGIN: " + <URL string of a disabled origin>
// * value: <Resource ID of the drive directory for the origin> (stripped)
const char kDatabaseVersionKey[] = "VERSION";
const char kSyncRootDirectoryKey[] = "SYNC_ROOT_DIR";
const char kDriveMetadataKeyPrefix[] = "METADATA: ";
const char kDriveBatchSyncOriginKeyPrefix[] = "BSYNC_ORIGIN: ";
const char kDriveIncrementalSyncOriginKeyPrefix[] = "ISYNC_ORIGIN: ";
const char kDriveDisabledOriginKeyPrefix[] = "DISABLED_ORIGIN: ";
leveldb::WriteBatch write_batch;
write_batch.Put(kDatabaseVersionKey, "2");
scoped_ptr<leveldb::Iterator> itr(db->NewIterator(leveldb::ReadOptions()));
for (itr->SeekToFirst(); itr->Valid(); itr->Next()) {
std::string key = itr->key().ToString();
// Strip resource id for the sync root directory.
if (StartsWithASCII(key, kSyncRootDirectoryKey, true)) {
write_batch.Put(key, RemoveWapiIdPrefix(itr->value().ToString()));
continue;
}
// Strip resource ids in the drive metadata.
if (StartsWithASCII(key, kDriveMetadataKeyPrefix, true)) {
DriveMetadata metadata;
bool success = metadata.ParseFromString(itr->value().ToString());
DCHECK(success);
metadata.set_resource_id(RemoveWapiIdPrefix(metadata.resource_id()));
std::string metadata_string;
metadata.SerializeToString(&metadata_string);
write_batch.Put(key, metadata_string);
continue;
}
// Deprecate legacy batch sync origin entries that are no longer needed.
if (StartsWithASCII(key, kDriveBatchSyncOriginKeyPrefix, true)) {
write_batch.Delete(key);
continue;
}
// Strip resource ids of the incremental sync origins.
if (StartsWithASCII(key, kDriveIncrementalSyncOriginKeyPrefix, true)) {
write_batch.Put(key, RemoveWapiIdPrefix(itr->value().ToString()));
continue;
}
// Strip resource ids of the disabled sync origins.
if (StartsWithASCII(key, kDriveDisabledOriginKeyPrefix, true)) {
write_batch.Put(key, RemoveWapiIdPrefix(itr->value().ToString()));
continue;
}
}
return LevelDBStatusToSyncStatusCode(
db->Write(leveldb::WriteOptions(), &write_batch));
}
} // namespace drive
} // namespace sync_file_system
| {'content_hash': '8bf45ebb9fc901e2df64c55bd92265ce', 'timestamp': '', 'source': 'github', 'line_count': 237, 'max_line_length': 77, 'avg_line_length': 35.78481012658228, 'alnum_prop': 0.6779860865463978, 'repo_name': 'windyuuy/opera', 'id': '96099c17bc25199028f0b0b44e51592db2ae9c68', 'size': '9043', 'binary': False, 'copies': '6', 'ref': 'refs/heads/master', 'path': 'chromium/src/chrome/browser/sync_file_system/drive/metadata_db_migration_util.cc', 'mode': '33188', 'license': 'bsd-3-clause', 'language': [{'name': 'ApacheConf', 'bytes': '25707'}, {'name': 'AppleScript', 'bytes': '6973'}, {'name': 'Assembly', 'bytes': '51642'}, {'name': 'Batchfile', 'bytes': '35942'}, {'name': 'C', 'bytes': '4303018'}, {'name': 'C#', 'bytes': '35203'}, {'name': 'C++', 'bytes': '207333360'}, {'name': 'CMake', 'bytes': '25089'}, {'name': 'CSS', 'bytes': '681256'}, {'name': 'Dart', 'bytes': '24294'}, {'name': 'Emacs Lisp', 'bytes': '25534'}, {'name': 'Groff', 'bytes': '5283'}, {'name': 'HTML', 'bytes': '10400943'}, {'name': 'IDL', 'bytes': '836'}, {'name': 'Java', 'bytes': '2821184'}, {'name': 'JavaScript', 'bytes': '14563996'}, {'name': 'Lua', 'bytes': '13749'}, {'name': 'Makefile', 'bytes': '55521'}, {'name': 'Objective-C', 'bytes': '1211523'}, {'name': 'Objective-C++', 'bytes': '6221908'}, {'name': 'PHP', 'bytes': '61320'}, {'name': 'Perl', 'bytes': '82949'}, {'name': 'Protocol Buffer', 'bytes': '280464'}, {'name': 'Python', 'bytes': '12627773'}, {'name': 'Rebol', 'bytes': '262'}, {'name': 'Ruby', 'bytes': '937'}, {'name': 'Scheme', 'bytes': '10604'}, {'name': 'Shell', 'bytes': '894814'}, {'name': 'VimL', 'bytes': '4953'}, {'name': 'XSLT', 'bytes': '418'}, {'name': 'nesC', 'bytes': '14650'}]} |
// ----------------------------------------------------------------------------------
//
// Copyright Microsoft Corporation
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
// http://www.apache.org/licenses/LICENSE-2.0
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
// ----------------------------------------------------------------------------------
using System;
using System.Web;
using System.Collections.Generic;
using System.Collections.Specialized;
using System.Linq;
using System.Management.Automation;
using System.Text;
using System.Threading.Tasks;
using Microsoft.Azure.Management.BackupServices.Models;
using MBS = Microsoft.Azure.Management.BackupServices;
using Microsoft.WindowsAzure.Commands.ServiceManagement.Model;
using Microsoft.Azure.Commands.AzureBackup.Properties;
using Microsoft.Azure.Commands.AzureBackup.Models;
using Microsoft.Azure.Commands.AzureBackup.Helpers;
using Microsoft.Azure.Management.BackupServices;
namespace Microsoft.Azure.Commands.AzureBackup.Cmdlets
{
/// <summary>
/// Enables reregistration of a machine container
/// </summary>
[Cmdlet(VerbsLifecycle.Enable, "AzureRMBackupContainerReregistration")]
public class EnableAzureRMBackupContainerReregistration : AzureBackupContainerCmdletBase
{
public override void ExecuteCmdlet()
{
ExecutionBlock(() =>
{
base.ExecuteCmdlet();
AzureBackupContainerType containerType = (AzureBackupContainerType)Enum.Parse(typeof(AzureBackupContainerType), Container.ContainerType);
switch (containerType)
{
case AzureBackupContainerType.Windows:
case AzureBackupContainerType.SCDPM:
AzureBackupClient.EnableMachineContainerReregistration(Container.ResourceGroupName, Container.ResourceName, Container.Id);
break;
default:
throw new ArgumentException(Resources.CannotEnableRegistration);
}
});
}
}
}
| {'content_hash': 'e6a3cab8d033bf519c543d67882fdf18', 'timestamp': '', 'source': 'github', 'line_count': 58, 'max_line_length': 153, 'avg_line_length': 42.86206896551724, 'alnum_prop': 0.6633145615446501, 'repo_name': 'jianghaolu/azure-powershell', 'id': '7fe6cc352f75f797604421424ab38181a8af297d', 'size': '2488', 'binary': False, 'copies': '4', 'ref': 'refs/heads/master', 'path': 'src/ResourceManager/AzureBackup/Commands.AzureBackup/Cmdlets/Container/EnableAzureRMBackupContainerReregistration.cs', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Batchfile', 'bytes': '15822'}, {'name': 'C#', 'bytes': '22432078'}, {'name': 'HTML', 'bytes': '209'}, {'name': 'JavaScript', 'bytes': '4979'}, {'name': 'PHP', 'bytes': '41'}, {'name': 'PowerShell', 'bytes': '1795127'}, {'name': 'Shell', 'bytes': '50'}]} |
class WDTypedResult;
//
// Result types for WebDataService.
//
typedef enum {
BOOL_RESULT = 1, // WDResult<bool>
KEYWORDS_RESULT, // WDResult<WDKeywordsResult>
INT64_RESULT, // WDResult<int64_t>
#if defined(OS_WIN) //
PASSWORD_IE7_RESULT, // WDResult<IE7PasswordInfo>
#endif //
WEB_APP_IMAGES, // WDResult<WDAppImagesResult>
TOKEN_RESULT, // WDResult<TokenResult>
AUTOFILL_VALUE_RESULT, // WDResult<std::vector<AutofillEntry>>
AUTOFILL_CLEANUP_RESULT, // WDResult<size_t>
AUTOFILL_CHANGES, // WDResult<std::vector<AutofillChange>>
AUTOFILL_PROFILE_RESULT, // WDResult<AutofillProfile>
AUTOFILL_PROFILES_RESULT, // WDResult<std::vector<
// std::unique_ptr<AutofillProfile>>>
AUTOFILL_CLOUDTOKEN_RESULT, // WDResult<std::vector<std::unique_ptr<
// CreditCardCloudTokenData>>>
AUTOFILL_CREDITCARD_RESULT, // WDResult<CreditCard>
AUTOFILL_CREDITCARDS_RESULT, // WDResult<std::vector<
// std::unique_ptr<CreditCard>>>
AUTOFILL_CUSTOMERDATA_RESULT, // WDResult<std::unique_ptr<
// PaymentsCustomerData>>
AUTOFILL_UPI_RESULT, // WDResult<std::string>
#if !defined(OS_IOS) //
PAYMENT_WEB_APP_MANIFEST, // WDResult<std::vector<
// mojom::WebAppManifestSectionPtr>>
PAYMENT_METHOD_MANIFEST, // WDResult<std::vector<std::string>>
#endif
} WDResultType;
//
// The top level class for a result.
//
class WEBDATA_EXPORT WDTypedResult {
public:
virtual ~WDTypedResult() {}
// Return the result type.
WDResultType GetType() const { return type_; }
protected:
explicit WDTypedResult(WDResultType type) : type_(type) {}
private:
WDResultType type_;
DISALLOW_COPY_AND_ASSIGN(WDTypedResult);
};
// A result containing one specific pointer or literal value.
template <class T>
class WDResult : public WDTypedResult {
public:
WDResult(WDResultType type, const T& v) : WDTypedResult(type), value_(v) {}
WDResult(WDResultType type, T&& v)
: WDTypedResult(type), value_(std::move(v)) {}
~WDResult() override {}
// Return a single value result.
const T& GetValue() const { return value_; }
T GetValue() { return std::move(value_); }
private:
T value_;
DISALLOW_COPY_AND_ASSIGN(WDResult);
};
#endif // COMPONENTS_WEBDATA_COMMON_WEB_DATA_RESULTS_H_
| {'content_hash': 'f77f5810da54a53b06c4e8cdf2fae602', 'timestamp': '', 'source': 'github', 'line_count': 74, 'max_line_length': 77, 'avg_line_length': 35.12162162162162, 'alnum_prop': 0.6063870719507503, 'repo_name': 'endlessm/chromium-browser', 'id': 'b69c6978174c8eaa4e2534522ba970fc71a34095', 'size': '3057', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'components/webdata/common/web_data_results.h', 'mode': '33188', 'license': 'bsd-3-clause', 'language': []} |
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<!-- NewPage -->
<html lang="en">
<head>
<!-- Generated by javadoc (1.8.0_60) on Mon Mar 28 17:57:43 AEST 2016 -->
<title>org.apache.river.api.io (River-Internet vtrunk API Documentation)</title>
<meta name="date" content="2016-03-28">
<link rel="stylesheet" type="text/css" href="../../../../../stylesheet.css" title="Style">
<script type="text/javascript" src="../../../../../script.js"></script>
</head>
<body>
<h1 class="bar"><a href="../../../../../org/apache/river/api/io/package-summary.html" target="classFrame">org.apache.river.api.io</a></h1>
<div class="indexContainer">
<h2 title="Interfaces">Interfaces</h2>
<ul title="Interfaces">
<li><a href="AtomicSerial.ReadObject.html" title="interface in org.apache.river.api.io" target="classFrame"><span class="interfaceName">AtomicSerial.ReadObject</span></a></li>
</ul>
<h2 title="Classes">Classes</h2>
<ul title="Classes">
<li><a href="AtomicExternal.Factory.html" title="class in org.apache.river.api.io" target="classFrame">AtomicExternal.Factory</a></li>
<li><a href="AtomicMarshalInputStream.html" title="class in org.apache.river.api.io" target="classFrame">AtomicMarshalInputStream</a></li>
<li><a href="AtomicMarshalOutputStream.html" title="class in org.apache.river.api.io" target="classFrame">AtomicMarshalOutputStream</a></li>
<li><a href="AtomicSerial.Factory.html" title="class in org.apache.river.api.io" target="classFrame">AtomicSerial.Factory</a></li>
<li><a href="AtomicSerial.GetArg.html" title="class in org.apache.river.api.io" target="classFrame">AtomicSerial.GetArg</a></li>
<li><a href="DeSerializationPermission.html" title="class in org.apache.river.api.io" target="classFrame">DeSerializationPermission</a></li>
<li><a href="UnsuportedOperationException.html" title="class in org.apache.river.api.io" target="classFrame">UnsuportedOperationException</a></li>
<li><a href="Valid.html" title="class in org.apache.river.api.io" target="classFrame">Valid</a></li>
</ul>
<h2 title="Enums">Enums</h2>
<ul title="Enums">
<li><a href="AtomicMarshalInputStream.Reference.html" title="enum in org.apache.river.api.io" target="classFrame">AtomicMarshalInputStream.Reference</a></li>
</ul>
<h2 title="Exceptions">Exceptions</h2>
<ul title="Exceptions">
<li><a href="AtomicException.html" title="class in org.apache.river.api.io" target="classFrame">AtomicException</a></li>
</ul>
<h2 title="Annotation Types">Annotation Types</h2>
<ul title="Annotation Types">
<li><a href="AtomicExternal.html" title="annotation in org.apache.river.api.io" target="classFrame">AtomicExternal</a></li>
<li><a href="AtomicSerial.html" title="annotation in org.apache.river.api.io" target="classFrame">AtomicSerial</a></li>
<li><a href="AtomicSerial.ReadInput.html" title="annotation in org.apache.river.api.io" target="classFrame">AtomicSerial.ReadInput</a></li>
<li><a href="Serializer.html" title="annotation in org.apache.river.api.io" target="classFrame">Serializer</a></li>
</ul>
</div>
</body>
</html>
| {'content_hash': 'f0a8e712d1e3aff916a8575064506b2d', 'timestamp': '', 'source': 'github', 'line_count': 46, 'max_line_length': 175, 'avg_line_length': 66.65217391304348, 'alnum_prop': 0.7292889758643183, 'repo_name': 'pfirmstone/JGDMS', 'id': '5fcef349a9973a478ccb2f41c5b8ba4953cd0cac', 'size': '3066', 'binary': False, 'copies': '2', 'ref': 'refs/heads/trunk', 'path': 'JGDMS/src/site/resources/old-static-site/doc/api/org/apache/river/api/io/package-frame.html', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'CSS', 'bytes': '38260'}, {'name': 'Groovy', 'bytes': '30510'}, {'name': 'HTML', 'bytes': '107806458'}, {'name': 'Java', 'bytes': '24863323'}, {'name': 'JavaScript', 'bytes': '1702'}, {'name': 'Makefile', 'bytes': '3032'}, {'name': 'Roff', 'bytes': '863'}, {'name': 'Shell', 'bytes': '68247'}]} |
angular
.module("avaliacao", ['ui.router', 'ui.bootstrap'])
.config(["$stateProvider", '$urlRouterProvider', "$locationProvider", routes]);
function routes($stateProvider, $urlRouterProvider, $locationProvider) {
$urlRouterProvider.otherwise('/');
/*
$locationProvider.html5Mode({
enabled: true,
requireBase: false
});
*/
$stateProvider
.state('home', {
url: '/',
templateUrl: 'templates/home.html'
})
.state('Login', {
url: '/Login',
templateUrl: 'templates/Login.html'
});
} | {'content_hash': '9c4f7f3803eb4e78baab83f40b44cbce', 'timestamp': '', 'source': 'github', 'line_count': 23, 'max_line_length': 83, 'avg_line_length': 26.652173913043477, 'alnum_prop': 0.5497553017944535, 'repo_name': 'MaikolSilva/desenvolvimento-web-ii', 'id': 'c9c10c93c6d0e4663b6ca952b7ac869693f2a4ee', 'size': '613', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'Avaliação/js/app.js', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'CSS', 'bytes': '803'}, {'name': 'HTML', 'bytes': '4267'}]} |
import './index';
import sinon from 'sinon';
import { expect } from 'chai';
import { $, $$ } from 'common-sk/modules/dom';
import fetchMock from 'fetch-mock';
import { GetTasksResponse } from '../json';
import {
singleResultCanDelete, singleResultNoDelete, resultSetOneItem, resultSetTwoItems,
} from './test_data';
import {
eventPromise,
setUpElementUnderTest,
} from '../../../infra-sk/modules/test_util';
describe('task-queue-sk', () => {
const newInstance = setUpElementUnderTest('task-queue-sk');
fetchMock.config.overwriteRoutes = false;
const loadTable = async () => {
const event = eventPromise('end-task');
const taskTableSk = newInstance();
await event;
return taskTableSk;
};
const loadTableWithReplies = async (replies: GetTasksResponse[]) => {
const kNumTaskQueries = 16;
const replyCount = replies.length;
expect(replyCount).to.be.most(kNumTaskQueries);
for (let i = 0; i < replyCount; ++i) {
fetchMock.postOnce('begin:/_/get_', replies[i]);
}
fetchMock.post('begin:/_/get_', 200, { repeat: kNumTaskQueries - replyCount });
return loadTable();
};
afterEach(() => {
// Check all mock fetches called at least once and reset.
expect(fetchMock.done()).to.be.true;
fetchMock.reset();
sinon.restore();
});
it('shows table entries', async () => {
// Return some results for 2 of the 16 task queries.
const table = await loadTableWithReplies([resultSetOneItem, resultSetTwoItems]);
// (3 items) * 6 columns
expect($('td', table).length).to.equal(18);
});
it('delete option shown', async () => {
const table = await loadTableWithReplies([singleResultCanDelete]);
expect($$('delete-icon-sk', table)).to.have.property('hidden', false);
});
it('delete option hidden', async () => {
const table = await loadTableWithReplies([singleResultNoDelete]);
expect($$('delete-icon-sk', table)).to.have.property('hidden', true);
});
it('delete flow works', async () => {
const table = await loadTableWithReplies([singleResultCanDelete]);
sinon.stub(window, 'confirm').returns(true);
sinon.stub(window, 'alert');
fetchMock.postOnce((url, options) => url.startsWith('/_/delete_') && options.body === JSON.stringify({ id: 1 }), 200);
($$('delete-icon-sk', table) as HTMLElement).click();
});
it('task details works', async () => {
const table = await loadTableWithReplies([resultSetOneItem]);
expect($$('.dialog-background', table)!.classList.value).to.include('hidden');
expect($$('.dialog-background', table)!.classList.value).to.include('hidden');
($$('.details', table) as HTMLElement).click();
expect($$('.dialog-background', table)!.classList.value).to.not.include('hidden');
});
});
| {'content_hash': '43fd414ca959907e65c5de1122d0626e', 'timestamp': '', 'source': 'github', 'line_count': 84, 'max_line_length': 122, 'avg_line_length': 33.04761904761905, 'alnum_prop': 0.6534582132564841, 'repo_name': 'google/skia-buildbot', 'id': 'c942c4cc023ef256228c8bfd83640f957a988700', 'size': '2776', 'binary': False, 'copies': '1', 'ref': 'refs/heads/main', 'path': 'ct/modules/task-queue-sk/task-queue-sk_test.ts', 'mode': '33188', 'license': 'bsd-3-clause', 'language': [{'name': 'Batchfile', 'bytes': '736'}, {'name': 'C', 'bytes': '3114'}, {'name': 'C++', 'bytes': '18072'}, {'name': 'CSS', 'bytes': '13967'}, {'name': 'Dockerfile', 'bytes': '18546'}, {'name': 'Go', 'bytes': '8744467'}, {'name': 'HTML', 'bytes': '790880'}, {'name': 'JavaScript', 'bytes': '1186449'}, {'name': 'Jupyter Notebook', 'bytes': '9165'}, {'name': 'Makefile', 'bytes': '75823'}, {'name': 'PowerShell', 'bytes': '15305'}, {'name': 'Python', 'bytes': '126773'}, {'name': 'SCSS', 'bytes': '128048'}, {'name': 'Shell', 'bytes': '232449'}, {'name': 'Starlark', 'bytes': '234929'}, {'name': 'TypeScript', 'bytes': '1568540'}]} |
#pragma once
#ifndef EL_READ_ASCII_HPP
#define EL_READ_ASCII_HPP
namespace El {
namespace read {
template<typename T>
inline void
Ascii( Matrix<T>& A, const string filename )
{
DEBUG_ONLY(CSE cse("read::Ascii"))
std::ifstream file( filename.c_str() );
if( !file.is_open() )
RuntimeError("Could not open ",filename);
// Walk through the file once to both count the number of rows and
// columns and to ensure that the number of columns is consistent
Int height=0, width=0;
string line;
while( std::getline( file, line ) )
{
std::stringstream lineStream( line );
Int numCols=0;
T value;
while( lineStream >> value ) ++numCols;
if( numCols != 0 )
{
if( numCols != width && width != 0 )
LogicError("Inconsistent number of columns");
else
width = numCols;
++height;
}
}
file.clear();
file.seekg(0,file.beg);
// Resize the matrix and then read it
A.Resize( height, width );
Int i=0;
while( std::getline( file, line ) )
{
std::stringstream lineStream( line );
Int j=0;
T value;
while( lineStream >> value )
{
A.Set( i, j, value );
++j;
}
++i;
}
}
template<typename T>
inline void
Ascii( AbstractDistMatrix<T>& A, const string filename )
{
DEBUG_ONLY(CSE cse("read::Ascii"))
std::ifstream file( filename.c_str() );
if( !file.is_open() )
RuntimeError("Could not open ",filename);
// Walk through the file once to both count the number of rows and
// columns and to ensure that the number of columns is consistent
Int height=0, width=0;
string line;
while( std::getline( file, line ) )
{
std::stringstream lineStream( line );
Int numCols=0;
T value;
while( lineStream >> value ) ++numCols;
if( numCols != 0 )
{
if( numCols != width && width != 0 )
LogicError("Inconsistent number of columns");
else
width = numCols;
++height;
}
}
file.clear();
file.seekg(0,file.beg);
// Resize the matrix and then read in our local portion
A.Resize( height, width );
Int i=0;
while( std::getline( file, line ) )
{
std::stringstream lineStream( line );
Int j=0;
T value;
while( lineStream >> value )
{
A.Set( i, j, value );
++j;
}
++i;
}
}
template<typename T>
inline void
Ascii( AbstractBlockDistMatrix<T>& A, const string filename )
{
DEBUG_ONLY(CSE cse("read::Ascii"))
std::ifstream file( filename.c_str() );
if( !file.is_open() )
RuntimeError("Could not open ",filename);
// Walk through the file once to both count the number of rows and
// columns and to ensure that the number of columns is consistent
Int height=0, width=0;
string line;
while( std::getline( file, line ) )
{
std::stringstream lineStream( line );
Int numCols=0;
T value;
while( lineStream >> value ) ++numCols;
if( numCols != 0 )
{
if( numCols != width && width != 0 )
LogicError("Inconsistent number of columns");
else
width = numCols;
++height;
}
}
file.clear();
file.seekg(0,file.beg);
// Resize the matrix and then read in our local portion
A.Resize( height, width );
Int i=0;
while( std::getline( file, line ) )
{
std::stringstream lineStream( line );
Int j=0;
T value;
while( lineStream >> value )
{
A.Set( i, j, value );
++j;
}
++i;
}
}
} // namespace read
} // namespace El
#endif // ifndef EL_READ_ASCII_HPP
| {'content_hash': 'be37942c1d5e3326e29d1fc221ad11c0', 'timestamp': '', 'source': 'github', 'line_count': 156, 'max_line_length': 70, 'avg_line_length': 25.185897435897434, 'alnum_prop': 0.5357597353016035, 'repo_name': 'justusc/Elemental', 'id': '20defeca2c500ce2defc4edba25439911e0df067', 'size': '4193', 'binary': False, 'copies': '2', 'ref': 'refs/heads/master', 'path': 'src/io/Read/Ascii.hpp', 'mode': '33188', 'license': 'bsd-3-clause', 'language': [{'name': 'C', 'bytes': '760573'}, {'name': 'C++', 'bytes': '7177017'}, {'name': 'CMake', 'bytes': '186926'}, {'name': 'Makefile', 'bytes': '333'}, {'name': 'Matlab', 'bytes': '13306'}, {'name': 'Python', 'bytes': '942707'}, {'name': 'Ruby', 'bytes': '1393'}, {'name': 'Shell', 'bytes': '1335'}, {'name': 'TeX', 'bytes': '23728'}]} |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>opam-website: Not compatible</title>
<link rel="shortcut icon" type="image/png" href="../../../../../favicon.png" />
<link href="../../../../../bootstrap.min.css" rel="stylesheet">
<link href="../../../../../bootstrap-custom.css" rel="stylesheet">
<link href="//maxcdn.bootstrapcdn.com/font-awesome/4.2.0/css/font-awesome.min.css" rel="stylesheet">
<script src="../../../../../moment.min.js"></script>
<!-- HTML5 Shim and Respond.js IE8 support of HTML5 elements and media queries -->
<!-- WARNING: Respond.js doesn't work if you view the page via file:// -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/html5shiv/3.7.2/html5shiv.min.js"></script>
<script src="https://oss.maxcdn.com/respond/1.4.2/respond.min.js"></script>
<![endif]-->
</head>
<body>
<div class="container">
<div class="navbar navbar-default" role="navigation">
<div class="container-fluid">
<div class="navbar-header">
<a class="navbar-brand" href="../../../../.."><i class="fa fa-lg fa-flag-checkered"></i> Coq bench</a>
</div>
<div id="navbar" class="collapse navbar-collapse">
<ul class="nav navbar-nav">
<li><a href="../..">clean / released</a></li>
<li class="active"><a href="">8.5.0 / opam-website - 1.2.1</a></li>
</ul>
</div>
</div>
</div>
<div class="article">
<div class="row">
<div class="col-md-12">
<a href="../..">« Up</a>
<h1>
opam-website
<small>
1.2.1
<span class="label label-info">Not compatible</span>
</small>
</h1>
<p><em><script>document.write(moment("2020-06-25 03:38:52 +0000", "YYYY-MM-DD HH:mm:ss Z").fromNow());</script> (2020-06-25 03:38:52 UTC)</em><p>
<h2>Context</h2>
<pre># Packages matching: installed
# Name # Installed # Synopsis
base-bigarray base
base-num base Num library distributed with the OCaml compiler
base-threads base
base-unix base
camlp5 7.12 Preprocessor-pretty-printer of OCaml
conf-findutils 1 Virtual package relying on findutils
coq 8.5.0 Formal proof management system.
num 0 The Num library for arbitrary-precision integer and rational arithmetic
ocaml 4.05.0 The OCaml compiler (virtual package)
ocaml-base-compiler 4.05.0 Official 4.05.0 release
ocaml-config 1 OCaml Switch Configuration
# opam file:
opam-version: "2.0"
maintainer: "[email protected]"
homepage: "https://github.com/clarus/coq-opam-website"
dev-repo: "git+https://github.com/clarus/coq-opam-website.git"
bug-reports: "https://github.com/clarus/coq-opam-website/issues"
authors: ["Guillaume Claret"]
license: "MIT"
build: [
["./configure.sh"]
[make "-j%{jobs}%"]
["sh" "-c" "cd extraction && make"]
]
depends: [
"ocaml"
"coq" {>= "8.4pl4"}
"coq-io" {>= "3.1.0"}
"coq-io-exception" {>= "1.0.0"}
"coq-io-system" {>= "2.3.0"}
"coq-list-string" {>= "2.1.0"}
]
synopsis: "Generation of a Coq website for OPAM: http://coq.io/opam/ "
url {
src: "https://github.com/coq-io/opam-website/archive/1.2.1.tar.gz"
checksum: "md5=8456933fe4380095c46240546fb2bad1"
}
</pre>
<h2>Lint</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Dry install</h2>
<p>Dry install with the current Coq version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam install -y --show-action coq-opam-website.1.2.1 coq.8.5.0</code></dd>
<dt>Return code</dt>
<dd>5120</dd>
<dt>Output</dt>
<dd><pre>[NOTE] Package coq is already installed (current version is 8.5.0).
The following dependencies couldn't be met:
- coq-opam-website -> coq-io-exception -> coq < 8.5~ -> ocaml < 4.03.0
base of this switch (use `--unlock-base' to force)
No solution found, exiting
</pre></dd>
</dl>
<p>Dry install without Coq/switch base, to test if the problem was incompatibility with the current Coq/OCaml version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam remove -y coq; opam install -y --show-action --unlock-base coq-opam-website.1.2.1</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Install dependencies</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>0 s</dd>
</dl>
<h2>Install</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>0 s</dd>
</dl>
<h2>Installation size</h2>
<p>No files were installed.</p>
<h2>Uninstall</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Missing removes</dt>
<dd>
none
</dd>
<dt>Wrong removes</dt>
<dd>
none
</dd>
</dl>
</div>
</div>
</div>
<hr/>
<div class="footer">
<p class="text-center">
<small>Sources are on <a href="https://github.com/coq-bench">GitHub</a>. © Guillaume Claret.</small>
</p>
</div>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
<script src="../../../../../bootstrap.min.js"></script>
</body>
</html>
| {'content_hash': '4ab1b2b0574a85e0ef395ea1715d806f', 'timestamp': '', 'source': 'github', 'line_count': 164, 'max_line_length': 157, 'avg_line_length': 40.707317073170735, 'alnum_prop': 0.5286099460754943, 'repo_name': 'coq-bench/coq-bench.github.io', 'id': '5e3ef27e413adbe420b32ced99dade95ddd562ac', 'size': '6678', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'clean/Linux-x86_64-4.05.0-2.0.6/released/8.5.0/opam-website/1.2.1.html', 'mode': '33188', 'license': 'mit', 'language': []} |
#include "unix/guts.h"
#include "Application.h"
#include "Clipboard.h"
#include "Icon.h"
#define WIN PComponent(application)-> handle
#define CF_NAME(x) (guts. clipboard_formats[(x)*3])
#define CF_TYPE(x) (guts. clipboard_formats[(x)*3+1])
#define CF_FORMAT(x) (guts. clipboard_formats[(x)*3+2])
#define CF_ASSIGN(i,a,b,c) CF_NAME(i)=(a);CF_TYPE(i)=(b);CF_FORMAT(i)=((Atom)c)
#define CF_32 (sizeof(long)*8) /* 32-bit properties are hacky */
Bool
prima_init_clipboard_subsystem(char * error_buf)
{
guts. clipboards = hash_create();
if ( !(guts. clipboard_formats = malloc( cfCOUNT * 3 * sizeof(Atom)))) {
sprintf( error_buf, "No memory");
return false;
}
guts. clipboard_formats_count = cfCOUNT;
#if (cfText != 0) || (cfBitmap != 1) || (cfUTF8 != 2)
#error broken clipboard type formats
#endif
CF_ASSIGN(cfText, XA_STRING, XA_STRING, 8);
CF_ASSIGN(cfUTF8, UTF8_STRING, UTF8_STRING, 8);
CF_ASSIGN(cfBitmap, XA_PIXMAP, XA_PIXMAP, CF_32);
CF_ASSIGN(cfTargets, CF_TARGETS, XA_ATOM, CF_32);
/* XXX - bitmaps and indexed pixmaps may have the associated colormap or pixel values
CF_ASSIGN(cfPalette, XA_COLORMAP, XA_ATOM, CF_32);
CF_ASSIGN(cfForeground, CF_FOREGROUND, CF_PIXEL, CF_32);
CF_ASSIGN(cfBackground, CF_BACKGROUND, CF_PIXEL, CF_32);
*/
guts. clipboard_event_timeout = 2000;
return true;
}
PList
apc_get_standard_clipboards( void)
{
PList l = plist_create( 3, 1);
if (!l) return nil;
list_add( l, (Handle)duplicate_string( "Primary"));
list_add( l, (Handle)duplicate_string( "Secondary"));
list_add( l, (Handle)duplicate_string( "Clipboard"));
return l;
}
Bool
apc_clipboard_create( Handle self)
{
PClipboard c = (PClipboard)self;
char *name, *x;
DEFCC;
XX-> selection = None;
name = x = duplicate_string( c-> name);
while (*x) {
*x = toupper(*x);
x++;
}
XX-> selection = XInternAtom( DISP, name, false);
free( name);
if ( hash_fetch( guts.clipboards, &XX->selection, sizeof(XX->selection))) {
warn("This clipboard is already present");
return false;
}
if ( !( XX-> internal = malloc( sizeof( ClipboardDataItem) * cfCOUNT))) {
warn("Not enough memory");
return false;
}
if ( !( XX-> external = malloc( sizeof( ClipboardDataItem) * cfCOUNT))) {
free( XX-> internal);
warn("Not enough memory");
return false;
}
bzero( XX-> internal, sizeof( ClipboardDataItem) * cfCOUNT);
bzero( XX-> external, sizeof( ClipboardDataItem) * cfCOUNT);
hash_store( guts.clipboards, &XX->selection, sizeof(XX->selection), (void*)self);
return true;
}
static void
clipboard_free_data( void * data, int size, Handle id)
{
if ( size <= 0) {
if ( size == 0 && data != nil) free( data);
return;
}
if ( id == cfBitmap) {
int i;
Pixmap * p = (Pixmap*) data;
for ( i = 0; i < size/sizeof(Pixmap); i++, p++)
if ( *p)
XFreePixmap( DISP, *p);
}
free( data);
}
/*
each clipboard type can be represented by a set of
X properties pairs, where each is X name and X type.
get_typename() returns such pairs by the index.
*/
static Atom
get_typename( Handle id, int index, Atom * type)
{
if ( type) *type = None;
switch ( id) {
case cfUTF8:
if ( index > 1) return None;
if ( index == 0) {
if ( type) *type = CF_TYPE(id);
return CF_NAME(id);
} else {
if ( type) *type = UTF8_MIME;
return UTF8_MIME;
}
case cfBitmap:
if ( index > 1) return None;
if ( index == 0) {
if ( type) *type = CF_TYPE(id);
return CF_NAME(id);
} else {
if ( type) *type = XA_BITMAP;
return XA_BITMAP;
}
case cfTargets:
if ( index > 1) return None;
if ( index == 0) {
if ( type) *type = CF_TYPE(id);
return CF_NAME(id);
} else {
if ( type) *type = CF_TARGETS;
return CF_NAME(id);
}
}
if ( index > 0) return None;
if ( type) *type = CF_TYPE(id);
return CF_NAME(id);
}
static void
clipboard_kill_item( PClipboardDataItem item, Handle id)
{
item += id;
clipboard_free_data( item-> data, item-> size, id);
item-> data = nil;
item-> size = 0;
item-> name = get_typename( id, 0, nil);
}
/*
Deletes a transfer record from pending xfer chain.
*/
static void
delete_xfer( PClipboardSysData cc, ClipboardXfer * xfer)
{
ClipboardXferKey key;
CLIPBOARD_XFER_KEY( key, xfer-> requestor, xfer-> property);
if ( guts. clipboard_xfers) {
IV refcnt;
hash_delete( guts. clipboard_xfers, key, sizeof( key), false);
refcnt = PTR2IV( hash_fetch( guts. clipboard_xfers, &xfer-> requestor, sizeof(XWindow)));
if ( --refcnt == 0) {
XSelectInput( DISP, xfer-> requestor, 0);
hash_delete( guts. clipboard_xfers, &xfer-> requestor, sizeof(XWindow), false);
} else {
if ( refcnt < 0) refcnt = 0;
hash_store( guts. clipboard_xfers, &xfer-> requestor, sizeof(XWindow), INT2PTR(void*, refcnt));
}
}
if ( cc-> xfers)
list_delete( cc-> xfers, ( Handle) xfer);
if ( xfer-> data_detached && xfer-> data_master)
clipboard_free_data( xfer-> data, xfer-> size, xfer-> id);
free( xfer);
}
Bool
apc_clipboard_destroy( Handle self)
{
DEFCC;
int i;
if (XX-> selection == None) return true;
if ( XX-> xfers) {
for ( i = 0; i < XX-> xfers-> count; i++)
delete_xfer( XX, ( ClipboardXfer*) XX-> xfers-> items[i]);
plist_destroy( XX-> xfers);
}
for ( i = 0; i < guts. clipboard_formats_count; i++) {
if ( XX-> external) clipboard_kill_item( XX-> external, i);
if ( XX-> internal) clipboard_kill_item( XX-> internal, i);
}
free( XX-> external);
free( XX-> internal);
hash_delete( guts.clipboards, &XX->selection, sizeof(XX->selection), false);
XX-> selection = None;
return true;
}
Bool
apc_clipboard_open( Handle self)
{
DEFCC;
if ( XX-> opened) return false;
XX-> opened = true;
if ( !XX-> inside_event) XX-> need_write = false;
return true;
}
Bool
apc_clipboard_close( Handle self)
{
DEFCC;
if ( !XX-> opened) return false;
XX-> opened = false;
/* check if UTF8 is present and Text is not, and downgrade */
if ( XX-> need_write &&
XX-> internal[cfUTF8]. size > 0 &&
XX-> internal[cfText]. size == 0) {
Byte * src = XX-> internal[cfUTF8]. data;
int len = utf8_length( src, src + XX-> internal[cfUTF8]. size);
if (( XX-> internal[cfText]. data = malloc( len))) {
STRLEN charlen;
U8 *dst;
dst = XX-> internal[cfText]. data;
XX-> internal[cfText]. size = len;
while ( len--) {
register UV u =
#if PERL_PATCHLEVEL >= 16
utf8_to_uvchr_buf( src, src + XX-> internal[cfUTF8]. size, &charlen);
#else
utf8_to_uvchr( src, &charlen)
#endif
;
*(dst++) = ( u < 0x7f) ? u : '?'; /* XXX employ $LANG and iconv() */
src += charlen;
}
}
}
if ( !XX-> inside_event) {
int i;
for ( i = 0; i < guts. clipboard_formats_count; i++)
clipboard_kill_item( XX-> external, i);
if ( XX-> need_write)
if ( XGetSelectionOwner( DISP, XX-> selection) != WIN)
XSetSelectionOwner( DISP, XX-> selection, WIN, CurrentTime);
}
return true;
}
/*
Detaches data for pending transfers from XX, so eventual changes
to XX->internal would not affect them. detach_xfers() should be
called before clipboard_kill_item(XX-> internal), otherwise
there's a chance of coredump.
*/
static void
detach_xfers( PClipboardSysData XX, Handle id, Bool clear_original_data)
{
int i, got_master = 0, got_anything = 0;
if ( !XX-> xfers) return;
for ( i = 0; i < XX-> xfers-> count; i++) {
ClipboardXfer * x = ( ClipboardXfer *) XX-> xfers-> items[i];
if ( x-> data_detached || x-> id != id) continue;
got_anything = 1;
if ( !got_master) {
x-> data_master = true;
got_master = 1;
}
x-> data_detached = true;
}
if ( got_anything && clear_original_data) {
XX-> internal[id]. data = nil;
XX-> internal[id]. size = 0;
XX-> internal[id]. name = get_typename( id, 0, nil);
}
}
Bool
apc_clipboard_clear( Handle self)
{
DEFCC;
int i;
for ( i = 0; i < guts. clipboard_formats_count; i++) {
detach_xfers( XX, i, true);
clipboard_kill_item( XX-> internal, i);
clipboard_kill_item( XX-> external, i);
}
if ( XX-> inside_event) {
XX-> need_write = true;
} else {
XWindow owner = XGetSelectionOwner( DISP, XX-> selection);
XX-> need_write = false;
if ( owner != None && owner != WIN)
XSetSelectionOwner( DISP, XX-> selection, None, CurrentTime);
}
return true;
}
typedef struct {
Atom selection;
long mask;
} SelectionProcData;
#define SELECTION_NOTIFY_MASK 1
#define PROPERTY_NOTIFY_MASK 2
static int
selection_filter( Display * disp, XEvent * ev, SelectionProcData * data)
{
switch ( ev-> type) {
case PropertyNotify:
return (data-> mask & PROPERTY_NOTIFY_MASK) && (data-> selection == ev-> xproperty. atom);
case SelectionRequest:
case SelectionClear:
case MappingNotify:
return true;
case SelectionNotify:
return (data-> mask & SELECTION_NOTIFY_MASK) && (data-> selection == ev-> xselection. selection);
case ClientMessage:
if ( ev-> xclient. window == WIN ||
ev-> xclient. window == guts. root ||
ev-> xclient. window == None) return true;
if ( hash_fetch( guts.windows, (void*)&ev-> xclient. window,
sizeof(ev-> xclient. window))) return false;
return true;
}
return false;
}
#define CFDATA_NONE 0
#define CFDATA_NOT_ACQUIRED (-1)
#define CFDATA_ERROR (-2)
#define RPS_OK 0
#define RPS_PARTIAL 1
#define RPS_NODATA 2
#define RPS_ERROR 3
static int
read_property( Atom property, Atom * type, int * format,
unsigned long * size, unsigned char ** data)
{
int ret = ( *size > 0) ? RPS_PARTIAL : RPS_ERROR;
unsigned char * prop, *a1;
unsigned long n, left, offs = 0, new_size, big_offs = *size;
XCHECKPOINT;
Cdebug("clipboard: read_property: %s\n", XGetAtomName(DISP, property));
while ( 1) {
if ( XGetWindowProperty( DISP, WIN, property,
offs, guts. limits. request_length - 4, false,
AnyPropertyType,
type, format, &n, &left, &prop) != Success) {
XDeleteProperty( DISP, WIN, property);
Cdebug("clipboard:fail\n");
return ret;
}
XCHECKPOINT;
Cdebug("clipboard: type=0x%x(%s) fmt=%d n=%d left=%d\n",
*type, XGetAtomName(DISP,*type), *format, n, left);
if ( *format == 32) *format = CF_32;
if ( *type == 0 ) return RPS_NODATA;
new_size = n * *format / 8;
if ( new_size > 0) {
if ( !( a1 = realloc( *data, big_offs + offs * 4 + new_size))) {
warn("Not enough memory: %ld bytes\n", offs * 4 + new_size);
XDeleteProperty( DISP, WIN, property);
XFree( prop);
return ret;
}
*data = a1;
memcpy( *data + big_offs + offs * 4, prop, new_size);
*size = big_offs + (offs * 4) + new_size;
if ( *size > INT_MAX) *size = INT_MAX;
offs += new_size / 4;
ret = RPS_PARTIAL;
}
XFree( prop);
if ( left <= 0 || *size == INT_MAX || n * *format == 0) break;
}
XDeleteProperty( DISP, WIN, property);
XCHECKPOINT;
return RPS_OK;
}
static Bool
query_datum( Handle self, Handle id, Atom query_target, Atom query_type)
{
DEFCC;
XEvent ev;
Atom type;
int format, rps;
SelectionProcData spd;
unsigned long size = 0, incr = 0, old_size, delay;
unsigned char * data;
struct timeval start_time, timeout;
/* init */
if ( query_target == None) return false;
data = malloc(0);
XX-> external[id]. size = CFDATA_ERROR;
gettimeofday( &start_time, nil);
XCHECKPOINT;
Cdebug("clipboard:convert %s from %08x\n", XGetAtomName( DISP, query_target), WIN);
XDeleteProperty( DISP, WIN, XX-> selection);
XConvertSelection( DISP, XX-> selection, query_target, XX-> selection, WIN, guts. last_time);
XFlush( DISP);
XCHECKPOINT;
/* wait for SelectionNotify */
spd. selection = XX-> selection;
spd. mask = SELECTION_NOTIFY_MASK;
while ( 1) {
XIfEvent( DISP, &ev, (XIfEventProcType)selection_filter, (char*)&spd);
if ( ev. type != SelectionNotify) {
prima_handle_event( &ev, nil);
continue;
}
if ( ev. xselection. property == None) goto FAIL;
Cdebug("clipboard:read SelectionNotify %s %s\n",
XGetAtomName(DISP, ev. xselection. property),
XGetAtomName(DISP, ev. xselection. target));
gettimeofday( &timeout, nil);
delay = 2 * (( timeout. tv_sec - start_time. tv_sec) * 1000 +
( timeout. tv_usec - start_time. tv_usec) / 1000) + guts. clipboard_event_timeout;
start_time = timeout;
if ( read_property( ev. xselection. property, &type, &format, &size, &data) > RPS_PARTIAL)
goto FAIL;
XFlush( DISP);
break;
}
XCHECKPOINT;
if ( type != XA_INCR) { /* ordinary, single-property selection */
if ( format != CF_FORMAT(id) || type != query_type) {
if ( format != CF_FORMAT(id))
Cdebug("clipboard: id=%d: formats mismatch: got %d, want %d\n", id, format, CF_FORMAT(id));
if ( type != query_type)
Cdebug("clipboard: id=%d: types mismatch: got %s, want %s\n", id,
XGetAtomName(DISP,type), XGetAtomName(DISP,query_type));
return false;
}
XX-> external[id]. size = size;
XX-> external[id]. data = data;
XX-> external[id]. name = query_target;
return true;
}
/* setup INCR */
if ( format != CF_32 || size < 4) goto FAIL;
incr = (unsigned long) *(( Atom*) data);
if ( incr == 0) goto FAIL;
size = 0;
spd. mask = PROPERTY_NOTIFY_MASK;
while ( 1) {
/* wait for PropertyNotify */
while ( XCheckIfEvent( DISP, &ev, (XIfEventProcType)selection_filter, (char*)&spd) == False) {
gettimeofday( &timeout, nil);
if ((( timeout. tv_sec - start_time. tv_sec) * 1000 +
( timeout. tv_usec - start_time. tv_usec) / 1000) > delay)
goto END_LOOP;
}
if ( ev. type != PropertyNotify) {
prima_handle_event( &ev, nil);
continue;
}
if ( ev. xproperty. state != PropertyNewValue) continue;
start_time = timeout;
old_size = size;
rps = read_property( ev. xproperty. atom, &type, &format, &size, &data);
XFlush( DISP);
if ( rps == RPS_NODATA) continue;
if ( rps == RPS_ERROR) goto FAIL;
if ( format != CF_FORMAT(id) || type != CF_TYPE(id)) return false;
if ( size > incr || /* read all in INCR */
rps == RPS_PARTIAL || /* failed somewhere */
( size == incr && old_size == size) /* wait for empty PropertyNotify otherwise */
) break;
}
END_LOOP:
XCHECKPOINT;
XX-> external[id]. size = size;
XX-> external[id]. data = data;
XX-> external[id]. name = query_target;
return true;
FAIL:
XCHECKPOINT;
free( data);
return false;
}
static Bool
query_data( Handle self, Handle id)
{
Atom name, type;
int index = 0;
while (( name = get_typename( id, index++, &type)) != None) {
if ( query_datum( self, id, name, type)) return true;
}
return false;
}
static Atom
find_atoms( Atom * data, int length, int id)
{
int i, index = 0;
Atom name;
while (( name = get_typename( id, index++, nil)) != None) {
for ( i = 0; i < length / sizeof(Atom); i++) {
if ( data[i] == name)
return name;
}
}
return None;
}
Bool
apc_clipboard_has_format( Handle self, Handle id)
{
DEFCC;
if ( id < 0 || id >= guts. clipboard_formats_count) return false;
if ( XX-> inside_event) {
return XX-> internal[id]. size > 0 || XX-> external[id]. size > 0;
} else {
if ( XX-> internal[id]. size > 0) return true;
if ( XX-> external[cfTargets]. size == 0) {
/* read TARGETS, which as array of ATOMs */
query_data( self, cfTargets);
if ( XX-> external[cfTargets].size > 0) {
int i, size = XX-> external[cfTargets].size;
Atom * data = ( Atom*)(XX-> external[cfTargets]. data);
Atom ret;
Cdebug("clipboard targets:");
for ( i = 0; i < size/4; i++)
Cdebug("%s\n", XGetAtomName( DISP, data[i]));
/* find our index for TARGETS[i], assign CFDATA_NOT_ACQUIRED to it */
for ( i = 0; i < guts. clipboard_formats_count; i++) {
if ( i == cfTargets) continue;
ret = find_atoms( data, size, i);
if ( ret != None && (
XX-> external[i]. size == 0 ||
XX-> external[i]. size == CFDATA_ERROR
)
) {
XX-> external[i]. size = CFDATA_NOT_ACQUIRED;
XX-> external[i]. name = ret;
}
}
if ( XX-> external[id]. size == 0 ||
XX-> external[id]. size == CFDATA_ERROR)
return false;
}
}
if ( XX-> external[id]. size > 0 ||
XX-> external[id]. size == CFDATA_NOT_ACQUIRED)
return true;
if ( XX-> external[id]. size == CFDATA_ERROR)
return false;
/* selection owner does not support TARGETS, so peek */
if ( XX-> external[cfTargets]. size == 0 && XX-> internal[id]. size == 0)
return query_data( self, id);
}
return false;
}
Bool
apc_clipboard_get_data( Handle self, Handle id, PClipboardDataRec c)
{
DEFCC;
STRLEN size;
unsigned char * data;
Atom name;
if ( id < 0 || id >= guts. clipboard_formats_count) return false;
if ( !XX-> inside_event) {
if ( XX-> internal[id]. size == 0) {
if ( XX-> external[id]. size == CFDATA_NOT_ACQUIRED) {
if ( !query_data( self, id)) return false;
}
if ( XX-> external[id]. size == CFDATA_ERROR) return false;
}
}
if ( XX-> internal[id]. size == CFDATA_ERROR) return false;
if ( XX-> internal[id]. size > 0) {
size = XX-> internal[id]. size;
data = XX-> internal[id]. data;
name = XX-> internal[id]. name;
} else {
size = XX-> external[id]. size;
data = XX-> external[id]. data;
name = XX-> external[id]. name;
}
if ( size == 0 || data == nil) return false;
switch ( id) {
case cfBitmap: {
Handle img = c-> image;
XWindow foo;
Pixmap px = *(( Pixmap*)( data));
unsigned int dummy, x, y, d;
int bar;
if ( !XGetGeometry( DISP, px, &foo, &bar, &bar, &x, &y, &dummy, &d))
return false;
CImage( img)-> create_empty( img, x, y, ( d == 1) ? imBW : guts. qdepth);
if ( !prima_std_query_image( img, px)) return false;
break;}
case cfText:
case cfUTF8: {
void * ret = malloc( size);
if ( !ret) {
warn("Not enough memory: %d bytes\n", (int)size);
return false;
}
memcpy( ret, data, size);
c-> data = ret;
c-> length = size;
break;}
default: {
void * ret = malloc( size);
if ( !ret) {
warn("Not enough memory: %d bytes\n", (int)size);
return false;
}
memcpy( ret, data, size);
c-> data = ( Byte * ) ret;
c-> length = size;
break;}
}
return true;
}
Bool
apc_clipboard_set_data( Handle self, Handle id, PClipboardDataRec c)
{
DEFCC;
if ( id < 0 || id >= guts. clipboard_formats_count) return false;
if ( id >= cfTargets && id < cfCOUNT ) return false;
detach_xfers( XX, id, true);
clipboard_kill_item( XX-> internal, id);
switch ( id) {
case cfBitmap: {
Pixmap px = prima_std_pixmap( c-> image, CACHE_LOW_RES);
if ( px) {
if ( !( XX-> internal[cfBitmap]. data = malloc( sizeof( px)))) {
XFreePixmap( DISP, px);
return false;
}
XX-> internal[cfBitmap]. size = sizeof(px);
memcpy( XX-> internal[cfBitmap]. data, &px, sizeof(px));
} else
return false;
break;}
default:
if ( !( XX-> internal[id]. data = malloc( c-> length)))
return false;
XX-> internal[id]. size = c-> length;
memcpy( XX-> internal[id]. data, c-> data, c-> length);
break;
}
XX-> need_write = true;
return true;
}
static Bool
expand_clipboards( Handle self, int keyLen, void * key, void * dummy)
{
DEFCC;
PClipboardDataItem f;
if ( !( f = realloc( XX-> internal,
sizeof( ClipboardDataItem) * guts. clipboard_formats_count))) {
guts. clipboard_formats_count--;
return true;
}
f[ guts. clipboard_formats_count-1].size = 0;
f[ guts. clipboard_formats_count-1].data = nil;
f[ guts. clipboard_formats_count-1].name = CF_NAME(guts. clipboard_formats_count-1);
XX-> internal = f;
if ( !( f = realloc( XX-> external,
sizeof( ClipboardDataItem) * guts. clipboard_formats_count))) {
guts. clipboard_formats_count--;
return true;
}
f[ guts. clipboard_formats_count-1].size = 0;
f[ guts. clipboard_formats_count-1].data = nil;
f[ guts. clipboard_formats_count-1].name = CF_NAME(guts. clipboard_formats_count-1);
XX-> external = f;
return false;
}
Handle
apc_clipboard_register_format( Handle self, const char* format)
{
int i;
Atom x = XInternAtom( DISP, format, false);
Atom *f;
for ( i = 0; i < guts. clipboard_formats_count; i++) {
if ( x == CF_NAME(i))
return i;
}
if ( !( f = realloc( guts. clipboard_formats,
sizeof( Atom) * 3 * ( guts. clipboard_formats_count + 1))))
return false;
guts. clipboard_formats = f;
CF_ASSIGN( guts. clipboard_formats_count, x, x, 8);
guts. clipboard_formats_count++;
if ( hash_first_that( guts. clipboards, (void*)expand_clipboards, nil, nil, nil))
return -1;
return guts. clipboard_formats_count - 1;
}
Bool
apc_clipboard_deregister_format( Handle self, Handle id)
{
return true;
}
ApiHandle
apc_clipboard_get_handle( Handle self)
{
return C(self)-> selection;
}
static Bool
delete_xfers( Handle self, int keyLen, void * key, XWindow * window)
{
DEFCC;
if ( XX-> xfers) {
int i;
for ( i = 0; i < XX-> xfers-> count; i++)
delete_xfer( XX, ( ClipboardXfer*) XX-> xfers-> items[i]);
}
hash_delete( guts. clipboard_xfers, window, sizeof( XWindow), false);
return false;
}
void
prima_handle_selection_event( XEvent *ev, XWindow win, Handle self)
{
XCHECKPOINT;
switch ( ev-> type) {
case SelectionRequest: {
XEvent xe;
int i, id = -1;
Atom prop = ev-> xselectionrequest. property,
target = ev-> xselectionrequest. target;
self = ( Handle) hash_fetch( guts. clipboards, &ev-> xselectionrequest. selection, sizeof( Atom));
guts. last_time = ev-> xselectionrequest. time;
xe. type = SelectionNotify;
xe. xselection. send_event = true;
xe. xselection. serial = ev-> xselectionrequest. serial;
xe. xselection. display = ev-> xselectionrequest. display;
xe. xselection. requestor = ev-> xselectionrequest. requestor;
xe. xselection. selection = ev-> xselectionrequest. selection;
xe. xselection. target = target;
xe. xselection. property = None;
xe. xselection. time = ev-> xselectionrequest. time;
Cdebug("from %08x %s at %s\n", ev-> xselectionrequest. requestor,
XGetAtomName( DISP, ev-> xselectionrequest. target),
XGetAtomName( DISP, ev-> xselectionrequest. property)
);
if ( self) {
PClipboardSysData CC = C(self);
Bool event = CC-> inside_event;
int format, utf8_mime = 0;
for ( i = 0; i < guts. clipboard_formats_count; i++) {
if ( xe. xselection. target == CC-> internal[i]. name) {
id = i;
break;
} else if ( i == cfUTF8 && xe. xselection. target == UTF8_MIME) {
id = i;
utf8_mime = 1;
break;
}
}
if ( id < 0) goto SEND_EMPTY;
for ( i = 0; i < guts. clipboard_formats_count; i++)
clipboard_kill_item( CC-> external, i);
CC-> target = xe. xselection. target;
CC-> need_write = false;
CC-> inside_event = true;
/* XXX cmSelection */
CC-> inside_event = event;
format = CF_FORMAT(id);
target = CF_TYPE( id);
if ( utf8_mime) target = UTF8_MIME;
if ( id == cfTargets) {
int count = 0, have_utf8 = 0;
Atom * ci;
for ( i = 0; i < guts. clipboard_formats_count; i++) {
if ( i != cfTargets && CC-> internal[i]. size > 0) {
count++;
if ( i == cfUTF8) {
count++;
have_utf8 = 1;
}
}
}
detach_xfers( CC, cfTargets, true);
clipboard_kill_item( CC-> internal, cfTargets);
if (( CC-> internal[cfTargets]. data = malloc( count * sizeof( Atom)))) {
CC-> internal[cfTargets]. size = count * sizeof( Atom);
ci = (Atom*)CC-> internal[cfTargets]. data;
for ( i = 0; i < guts. clipboard_formats_count; i++)
if ( i != cfTargets && CC-> internal[i]. size > 0)
*(ci++) = CF_NAME(i);
if ( have_utf8)
*(ci++) = UTF8_MIME;
}
}
if ( CC-> internal[id]. size > 0) {
Atom incr;
int mode = PropModeReplace;
unsigned char * data = CC-> internal[id]. data;
unsigned long size = CC-> internal[id]. size * 8 / format;
if ( CC-> internal[id]. size > guts. limits. request_length - 4) {
int ok = 0;
int reqlen = guts. limits. request_length - 4;
/* INCR */
if ( !guts. clipboard_xfers)
guts. clipboard_xfers = hash_create();
if ( !CC-> xfers)
CC-> xfers = plist_create( 1, 1);
if ( CC-> xfers && guts. clipboard_xfers) {
ClipboardXfer * x = malloc( sizeof( ClipboardXfer));
if ( x) {
IV refcnt;
ClipboardXferKey key;
bzero( x, sizeof( ClipboardXfer));
list_add( CC-> xfers, ( Handle) x);
x-> size = CC-> internal[id]. size;
x-> data = CC-> internal[id]. data;
x-> blocks = ( x-> size / reqlen ) + ( x-> size % reqlen) ? 1 : 0;
x-> requestor = xe. xselection. requestor;
x-> property = prop;
x-> target = xe. xselection. target;
x-> self = self;
x-> format = format;
x-> id = id;
gettimeofday( &x-> time, nil);
CLIPBOARD_XFER_KEY( key, x-> requestor, x-> property);
hash_store( guts. clipboard_xfers, key, sizeof(key), (void*) x);
refcnt = PTR2IV( hash_fetch( guts. clipboard_xfers, &x-> requestor, sizeof( XWindow)));
if ( refcnt++ == 0)
XSelectInput( DISP, x-> requestor, PropertyChangeMask|StructureNotifyMask);
hash_store( guts. clipboard_xfers, &x-> requestor, sizeof(XWindow), INT2PTR( void*, refcnt));
format = CF_32;
size = 1;
incr = ( Atom) CC-> internal[id]. size;
data = ( unsigned char*) &incr;
ok = 1;
target = XA_INCR;
Cdebug("clpboard: init INCR for %08x %d\n", x-> requestor, x-> property);
}
}
if ( !ok) size = reqlen;
}
if ( format == CF_32) format = 32;
XChangeProperty(
xe. xselection. display,
xe. xselection. requestor,
prop, target, format, mode, data, size);
Cdebug("clipboard: store prop %s\n", XGetAtomName( DISP, prop));
xe. xselection. property = prop;
}
/* content of PIXMAP or BITMAP is seemingly gets invalidated
after the selection transfer, unlike the string data format */
if ( id == cfBitmap) {
bzero( CC-> internal[id].data, CC-> internal[id].size);
bzero( CC-> external[id].data, CC-> external[id].size);
clipboard_kill_item( CC-> internal, id);
clipboard_kill_item( CC-> external, id);
}
}
SEND_EMPTY:
XSendEvent( xe.xselection.display, xe.xselection.requestor, false, 0, &xe);
XFlush( DISP);
Cdebug("clipboard:id %d, SelectionNotify to %08x , %s %s\n", id, xe.xselection.requestor,
XGetAtomName( DISP, xe. xselection. property),
XGetAtomName( DISP, xe. xselection. target));
} break;
case SelectionClear:
guts. last_time = ev-> xselectionclear. time;
if ( XGetSelectionOwner( DISP, ev-> xselectionclear. selection) != WIN) {
Handle c = ( Handle) hash_fetch( guts. clipboards,
&ev-> xselectionclear. selection, sizeof( Atom));
guts. last_time = ev-> xselectionclear. time;
if (c) {
int i;
C(c)-> selection_owner = nilHandle;
for ( i = 0; i < guts. clipboard_formats_count; i++) {
detach_xfers( C(c), i, true);
clipboard_kill_item( C(c)-> external, i);
clipboard_kill_item( C(c)-> internal, i);
}
}
}
break;
case PropertyNotify:
if ( ev-> xproperty. state == PropertyDelete) {
unsigned long offs, size, reqlen = guts. limits. request_length - 4, format;
ClipboardXfer * x = ( ClipboardXfer *) self;
PClipboardSysData CC = C(x-> self);
offs = x-> offset * reqlen;
if ( offs >= x-> size) { /* clear termination */
size = 0;
offs = 0;
} else {
size = x-> size - offs;
if ( size > reqlen) size = reqlen;
}
Cdebug("clipboard: put %d %d in %08x %d\n", x-> offset, size, x-> requestor, x-> property);
if ( x-> format > 8) size /= 2;
if ( x-> format > 16) size /= 2;
format = ( x-> format == CF_32) ? 32 : x-> format;
XChangeProperty( DISP, x-> requestor, x-> property, x-> target,
format, PropModeReplace,
x-> data + offs, size);
XFlush( DISP);
x-> offset++;
if ( size == 0) delete_xfer( CC, x);
}
break;
case DestroyNotify:
Cdebug("clipboard: destroy xfers at %08x\n", ev-> xdestroywindow. window);
hash_first_that( guts. clipboards, (void*)delete_xfers, (void*) &ev-> xdestroywindow. window, nil, nil);
XFlush( DISP);
break;
}
XCHECKPOINT;
}
| {'content_hash': 'b524844abd8f17aad1bb619d75502ef3', 'timestamp': '', 'source': 'github', 'line_count': 1008, 'max_line_length': 114, 'avg_line_length': 31.44047619047619, 'alnum_prop': 0.541713997223274, 'repo_name': 'run4flat/Primo', 'id': '2131edac613fcff0a4faf7d06534c35e2f2a0cc8', 'size': '33097', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'unix/apc_clipboard.c', 'mode': '33188', 'license': 'bsd-2-clause', 'language': [{'name': 'Apex', 'bytes': '56518'}, {'name': 'C', 'bytes': '1660328'}, {'name': 'Perl', 'bytes': '268749'}]} |
package scaleio
import (
"fmt"
"os"
"path/filepath"
"strconv"
"strings"
"k8s.io/klog/v2"
"k8s.io/mount-utils"
utilstrings "k8s.io/utils/strings"
api "k8s.io/api/core/v1"
"k8s.io/apimachinery/pkg/api/resource"
meta "k8s.io/apimachinery/pkg/apis/meta/v1"
"k8s.io/apimachinery/pkg/types"
"k8s.io/apimachinery/pkg/util/uuid"
volumehelpers "k8s.io/cloud-provider/volume/helpers"
"k8s.io/kubernetes/pkg/volume"
"k8s.io/kubernetes/pkg/volume/util"
)
type sioVolume struct {
sioMgr *sioMgr
plugin *sioPlugin
pod *api.Pod
podUID types.UID
spec *volume.Spec
secretName string
secretNamespace string
volSpecName string
volName string
readOnly bool
fsType string
options volume.VolumeOptions
configData map[string]string
volume.MetricsNil
}
const (
minimumVolumeSizeGiB = 8
)
// *******************
// volume.Volume Impl
var _ volume.Volume = &sioVolume{}
// GetPath returns the path where the volume will be mounted.
func (v *sioVolume) GetPath() string {
return v.plugin.host.GetPodVolumeDir(
v.podUID,
utilstrings.EscapeQualifiedName(sioPluginName),
v.volSpecName)
}
// *************
// Mounter Impl
// *************
var _ volume.Mounter = &sioVolume{}
// CanMount checks to verify that the volume can be mounted prior to Setup.
// A nil error indicates that the volume is ready for mounitnig.
func (v *sioVolume) CanMount() error {
return nil
}
func (v *sioVolume) SetUp(mounterArgs volume.MounterArgs) error {
return v.SetUpAt(v.GetPath(), mounterArgs)
}
// SetUp bind mounts the disk global mount to the volume path.
func (v *sioVolume) SetUpAt(dir string, mounterArgs volume.MounterArgs) error {
v.plugin.volumeMtx.LockKey(v.volSpecName)
defer v.plugin.volumeMtx.UnlockKey(v.volSpecName)
klog.V(4).Info(log("setting up volume for PV.spec %s", v.volSpecName))
if err := v.setSioMgr(); err != nil {
klog.Error(log("setup failed to create scalio manager: %v", err))
return err
}
mounter := v.plugin.host.GetMounter(v.plugin.GetPluginName())
notDevMnt, err := mounter.IsLikelyNotMountPoint(dir)
if err != nil && !os.IsNotExist(err) {
klog.Error(log("IsLikelyNotMountPoint test failed for dir %v", dir))
return err
}
if !notDevMnt {
klog.V(4).Info(log("skipping setup, dir %s already a mount point", v.volName))
return nil
}
// should multiple-mapping be enabled
enableMultiMaps := false
isROM := false
if v.spec.PersistentVolume != nil {
ams := v.spec.PersistentVolume.Spec.AccessModes
for _, am := range ams {
if am == api.ReadOnlyMany {
enableMultiMaps = true
isROM = true
}
}
}
klog.V(4).Info(log("multiple mapping enabled = %v", enableMultiMaps))
volName := v.volName
devicePath, err := v.sioMgr.AttachVolume(volName, enableMultiMaps)
if err != nil {
klog.Error(log("setup of volume %v: %v", v.volSpecName, err))
return err
}
options := []string{}
switch {
default:
options = append(options, "rw")
case isROM && !v.readOnly:
options = append(options, "rw")
case isROM:
options = append(options, "ro")
case v.readOnly:
options = append(options, "ro")
}
klog.V(4).Info(log("mounting device %s -> %s", devicePath, dir))
if err := os.MkdirAll(dir, 0750); err != nil {
klog.Error(log("failed to create dir %#v: %v", dir, err))
return err
}
klog.V(4).Info(log("setup created mount point directory %s", dir))
diskMounter := util.NewSafeFormatAndMountFromHost(v.plugin.GetPluginName(), v.plugin.host)
err = diskMounter.FormatAndMount(devicePath, dir, v.fsType, options)
if err != nil {
klog.Error(log("mount operation failed during setup: %v", err))
if err := os.Remove(dir); err != nil && !os.IsNotExist(err) {
klog.Error(log("failed to remove dir %s during a failed mount at setup: %v", dir, err))
return err
}
return err
}
if !v.readOnly && mounterArgs.FsGroup != nil {
klog.V(4).Info(log("applying value FSGroup ownership"))
volume.SetVolumeOwnership(v, mounterArgs.FsGroup, mounterArgs.FSGroupChangePolicy, util.FSGroupCompleteHook(v.plugin.GetPluginName()))
}
klog.V(4).Info(log("successfully setup PV %s: volume %s mapped as %s mounted at %s", v.volSpecName, v.volName, devicePath, dir))
return nil
}
func (v *sioVolume) GetAttributes() volume.Attributes {
return volume.Attributes{
ReadOnly: v.readOnly,
Managed: !v.readOnly,
SupportsSELinux: true,
}
}
// **********************
// volume.Unmounter Impl
// *********************
var _ volume.Unmounter = &sioVolume{}
// TearDownAt unmounts the bind mount
func (v *sioVolume) TearDown() error {
return v.TearDownAt(v.GetPath())
}
// TearDown unmounts and remove the volume
func (v *sioVolume) TearDownAt(dir string) error {
v.plugin.volumeMtx.LockKey(v.volSpecName)
defer v.plugin.volumeMtx.UnlockKey(v.volSpecName)
mounter := v.plugin.host.GetMounter(v.plugin.GetPluginName())
dev, _, err := mount.GetDeviceNameFromMount(mounter, dir)
if err != nil {
klog.Errorf(log("failed to get reference count for volume: %s", dir))
return err
}
klog.V(4).Info(log("attempting to unmount %s", dir))
if err := mount.CleanupMountPoint(dir, mounter, false); err != nil {
klog.Error(log("teardown failed while unmounting dir %s: %v ", dir, err))
return err
}
klog.V(4).Info(log("dir %s unmounted successfully", dir))
// detach/unmap
kvh, ok := v.plugin.host.(volume.KubeletVolumeHost)
if !ok {
return fmt.Errorf("plugin volume host does not implement KubeletVolumeHost interface")
}
hu := kvh.GetHostUtil()
deviceBusy, err := hu.DeviceOpened(dev)
if err != nil {
klog.Error(log("teardown unable to get status for device %s: %v", dev, err))
return err
}
// Detach volume from node:
// use "last attempt wins" strategy to detach volume from node
// only allow volume to detach when it is not busy (not being used by other pods)
if !deviceBusy {
klog.V(4).Info(log("teardown is attempting to detach/unmap volume for PV %s", v.volSpecName))
if err := v.resetSioMgr(); err != nil {
klog.Error(log("teardown failed, unable to reset scalio mgr: %v", err))
}
volName := v.volName
if err := v.sioMgr.DetachVolume(volName); err != nil {
klog.Warning(log("warning: detaching failed for volume %s: %v", volName, err))
return nil
}
klog.V(4).Infof(log("teardown of volume %v detached successfully", volName))
}
return nil
}
// ********************
// volume.Deleter Impl
// ********************
var _ volume.Deleter = &sioVolume{}
func (v *sioVolume) Delete() error {
klog.V(4).Info(log("deleting pvc %s", v.volSpecName))
if err := v.setSioMgrFromSpec(); err != nil {
klog.Error(log("delete failed while setting sio manager: %v", err))
return err
}
err := v.sioMgr.DeleteVolume(v.volName)
if err != nil {
klog.Error(log("failed to delete volume %s: %v", v.volName, err))
return err
}
klog.V(4).Info(log("successfully deleted PV %s with volume %s", v.volSpecName, v.volName))
return nil
}
// ************************
// volume.Provisioner Impl
// ************************
var _ volume.Provisioner = &sioVolume{}
func (v *sioVolume) Provision(selectedNode *api.Node, allowedTopologies []api.TopologySelectorTerm) (*api.PersistentVolume, error) {
klog.V(4).Info(log("attempting to dynamically provision pvc %v", v.options.PVC.Name))
if !util.AccessModesContainedInAll(v.plugin.GetAccessModes(), v.options.PVC.Spec.AccessModes) {
return nil, fmt.Errorf("invalid AccessModes %v: only AccessModes %v are supported", v.options.PVC.Spec.AccessModes, v.plugin.GetAccessModes())
}
if util.CheckPersistentVolumeClaimModeBlock(v.options.PVC) {
return nil, fmt.Errorf("%s does not support block volume provisioning", v.plugin.GetPluginName())
}
// setup volume attrributes
genName := v.generateName("k8svol", 11)
capacity := v.options.PVC.Spec.Resources.Requests[api.ResourceName(api.ResourceStorage)]
volSizeGiB, err := volumehelpers.RoundUpToGiB(capacity)
if err != nil {
return nil, err
}
if volSizeGiB < minimumVolumeSizeGiB {
volSizeGiB = minimumVolumeSizeGiB
klog.V(4).Info(log("capacity less than 8Gi found, adjusted to %dGi", volSizeGiB))
}
// create sio manager
if err := v.setSioMgrFromConfig(); err != nil {
klog.Error(log("provision failed while setting up sio mgr: %v", err))
return nil, err
}
// create volume
volName := genName
vol, err := v.sioMgr.CreateVolume(volName, volSizeGiB)
if err != nil {
klog.Error(log("provision failed while creating volume: %v", err))
return nil, err
}
// prepare data for pv
v.configData[confKey.volumeName] = volName
sslEnabled, err := strconv.ParseBool(v.configData[confKey.sslEnabled])
if err != nil {
klog.Warning(log("failed to parse parameter sslEnabled, setting to false"))
sslEnabled = false
}
readOnly, err := strconv.ParseBool(v.configData[confKey.readOnly])
if err != nil {
klog.Warning(log("failed to parse parameter readOnly, setting it to false"))
readOnly = false
}
// describe created pv
pvName := genName
pv := &api.PersistentVolume{
ObjectMeta: meta.ObjectMeta{
Name: pvName,
Namespace: v.options.PVC.Namespace,
Labels: map[string]string{},
Annotations: map[string]string{
util.VolumeDynamicallyCreatedByKey: "scaleio-dynamic-provisioner",
},
},
Spec: api.PersistentVolumeSpec{
PersistentVolumeReclaimPolicy: v.options.PersistentVolumeReclaimPolicy,
AccessModes: v.options.PVC.Spec.AccessModes,
Capacity: api.ResourceList{
api.ResourceName(api.ResourceStorage): resource.MustParse(
fmt.Sprintf("%dGi", volSizeGiB),
),
},
PersistentVolumeSource: api.PersistentVolumeSource{
ScaleIO: &api.ScaleIOPersistentVolumeSource{
Gateway: v.configData[confKey.gateway],
SSLEnabled: sslEnabled,
SecretRef: &api.SecretReference{Name: v.secretName, Namespace: v.secretNamespace},
System: v.configData[confKey.system],
ProtectionDomain: v.configData[confKey.protectionDomain],
StoragePool: v.configData[confKey.storagePool],
StorageMode: v.configData[confKey.storageMode],
VolumeName: volName,
FSType: v.configData[confKey.fsType],
ReadOnly: readOnly,
},
},
},
}
if len(v.options.PVC.Spec.AccessModes) == 0 {
pv.Spec.AccessModes = v.plugin.GetAccessModes()
}
klog.V(4).Info(log("provisioner created pv %v and volume %s successfully", pvName, vol.Name))
return pv, nil
}
// setSioMgr creates scaleio mgr from cached config data if found
// otherwise, setups new config data and create mgr
func (v *sioVolume) setSioMgr() error {
klog.V(4).Info(log("setting up sio mgr for spec %s", v.volSpecName))
podDir := v.plugin.host.GetPodPluginDir(v.podUID, sioPluginName)
configName := filepath.Join(podDir, sioConfigFileName)
if v.sioMgr == nil {
configData, err := loadConfig(configName) // try to load config if exist
if err != nil {
if !os.IsNotExist(err) {
klog.Error(log("failed to load config %s : %v", configName, err))
return err
}
klog.V(4).Info(log("previous config file not found, creating new one"))
// prepare config data
configData = make(map[string]string)
mapVolumeSpec(configData, v.spec)
// additional config data
configData[confKey.secretNamespace] = v.secretNamespace
configData[confKey.secretName] = v.secretName
configData[confKey.volSpecName] = v.volSpecName
if err := validateConfigs(configData); err != nil {
klog.Error(log("config setup failed: %s", err))
return err
}
// persist config
if err := saveConfig(configName, configData); err != nil {
klog.Error(log("failed to save config data: %v", err))
return err
}
}
// merge in secret
if err := attachSecret(v.plugin, v.secretNamespace, configData); err != nil {
klog.Error(log("failed to load secret: %v", err))
return err
}
// merge in Sdc Guid label value
if err := attachSdcGUID(v.plugin, configData); err != nil {
klog.Error(log("failed to retrieve sdc guid: %v", err))
return err
}
mgr, err := newSioMgr(configData, v.plugin.host, v.plugin.host.GetExec(v.plugin.GetPluginName()))
if err != nil {
klog.Error(log("failed to reset sio manager: %v", err))
return err
}
v.sioMgr = mgr
}
return nil
}
// resetSioMgr creates scaleio manager from existing (cached) config data
func (v *sioVolume) resetSioMgr() error {
podDir := v.plugin.host.GetPodPluginDir(v.podUID, sioPluginName)
configName := filepath.Join(podDir, sioConfigFileName)
if v.sioMgr == nil {
// load config data from disk
configData, err := loadConfig(configName)
if err != nil {
klog.Error(log("failed to load config data: %v", err))
return err
}
v.secretName = configData[confKey.secretName]
v.secretNamespace = configData[confKey.secretNamespace]
v.volName = configData[confKey.volumeName]
v.volSpecName = configData[confKey.volSpecName]
// attach secret
if err := attachSecret(v.plugin, v.secretNamespace, configData); err != nil {
klog.Error(log("failed to load secret: %v", err))
return err
}
// merge in Sdc Guid label value
if err := attachSdcGUID(v.plugin, configData); err != nil {
klog.Error(log("failed to retrieve sdc guid: %v", err))
return err
}
mgr, err := newSioMgr(configData, v.plugin.host, v.plugin.host.GetExec(v.plugin.GetPluginName()))
if err != nil {
klog.Error(log("failed to reset scaleio mgr: %v", err))
return err
}
v.sioMgr = mgr
}
return nil
}
// setSioFromConfig sets up scaleio mgr from an available config data map
// designed to be called from dynamic provisioner
func (v *sioVolume) setSioMgrFromConfig() error {
klog.V(4).Info(log("setting scaleio mgr from available config"))
if v.sioMgr == nil {
applyConfigDefaults(v.configData)
v.configData[confKey.volSpecName] = v.volSpecName
if err := validateConfigs(v.configData); err != nil {
klog.Error(log("config data setup failed: %s", err))
return err
}
// copy config and attach secret
data := map[string]string{}
for k, v := range v.configData {
data[k] = v
}
if err := attachSecret(v.plugin, v.secretNamespace, data); err != nil {
klog.Error(log("failed to load secret: %v", err))
return err
}
mgr, err := newSioMgr(data, v.plugin.host, v.plugin.host.GetExec(v.plugin.GetPluginName()))
if err != nil {
klog.Error(log("failed while setting scaleio mgr from config: %v", err))
return err
}
v.sioMgr = mgr
}
return nil
}
// setSioMgrFromSpec sets the scaleio manager from a spec object.
// The spec may be complete or incomplete depending on lifecycle phase.
func (v *sioVolume) setSioMgrFromSpec() error {
klog.V(4).Info(log("setting sio manager from spec"))
if v.sioMgr == nil {
// get config data form spec volume source
configData := map[string]string{}
mapVolumeSpec(configData, v.spec)
// additional config
configData[confKey.secretNamespace] = v.secretNamespace
configData[confKey.secretName] = v.secretName
configData[confKey.volSpecName] = v.volSpecName
if err := validateConfigs(configData); err != nil {
klog.Error(log("config setup failed: %s", err))
return err
}
// attach secret object to config data
if err := attachSecret(v.plugin, v.secretNamespace, configData); err != nil {
klog.Error(log("failed to load secret: %v", err))
return err
}
mgr, err := newSioMgr(configData, v.plugin.host, v.plugin.host.GetExec(v.plugin.GetPluginName()))
if err != nil {
klog.Error(log("failed to reset sio manager: %v", err))
return err
}
v.sioMgr = mgr
}
return nil
}
func (v *sioVolume) generateName(prefix string, size int) string {
return fmt.Sprintf("%s-%s", prefix, strings.Replace(string(uuid.NewUUID()), "-", "", -1)[0:size])
}
| {'content_hash': '4c549c5effcc93c5f43f8883f30e5f82', 'timestamp': '', 'source': 'github', 'line_count': 516, 'max_line_length': 144, 'avg_line_length': 30.606589147286822, 'alnum_prop': 0.6855568922940544, 'repo_name': 'kevensen/kubernetes', 'id': '23328428ca084e5ca2ccc2b31ed032a818c6f6bc', 'size': '16362', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'pkg/volume/scaleio/sio_volume.go', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'C', 'bytes': '998'}, {'name': 'Go', 'bytes': '27145101'}, {'name': 'HTML', 'bytes': '1193990'}, {'name': 'Makefile', 'bytes': '62023'}, {'name': 'Nginx', 'bytes': '1013'}, {'name': 'Protocol Buffer', 'bytes': '242445'}, {'name': 'Python', 'bytes': '34630'}, {'name': 'SaltStack', 'bytes': '55886'}, {'name': 'Shell', 'bytes': '1412739'}]} |
extern "Java"
{
namespace javax
{
namespace security
{
namespace auth
{
namespace login
{
class FailedLoginException;
}
}
}
}
}
class javax::security::auth::login::FailedLoginException : public ::javax::security::auth::login::LoginException
{
public:
FailedLoginException();
FailedLoginException(::java::lang::String *);
private:
static const jlong serialVersionUID = 802556922354616286LL;
public:
static ::java::lang::Class class$;
};
#endif // __javax_security_auth_login_FailedLoginException__
| {'content_hash': '6eb54b5a6e9da1ede7f8561a7b9d5db9', 'timestamp': '', 'source': 'github', 'line_count': 30, 'max_line_length': 112, 'avg_line_length': 19.333333333333332, 'alnum_prop': 0.6517241379310345, 'repo_name': 'the-linix-project/linix-kernel-source', 'id': '014a472c6d9e21351ef149f970f53ab5a268e814', 'size': '837', 'binary': False, 'copies': '160', 'ref': 'refs/heads/master', 'path': 'gccsrc/gcc-4.7.2/libjava/javax/security/auth/login/FailedLoginException.h', 'mode': '33188', 'license': 'bsd-2-clause', 'language': [{'name': 'Ada', 'bytes': '38139979'}, {'name': 'Assembly', 'bytes': '3723477'}, {'name': 'Awk', 'bytes': '83739'}, {'name': 'C', 'bytes': '103607293'}, {'name': 'C#', 'bytes': '55726'}, {'name': 'C++', 'bytes': '38577421'}, {'name': 'CLIPS', 'bytes': '6933'}, {'name': 'CSS', 'bytes': '32588'}, {'name': 'Emacs Lisp', 'bytes': '13451'}, {'name': 'FORTRAN', 'bytes': '4294984'}, {'name': 'GAP', 'bytes': '13089'}, {'name': 'Go', 'bytes': '11277335'}, {'name': 'Haskell', 'bytes': '2415'}, {'name': 'Java', 'bytes': '45298678'}, {'name': 'JavaScript', 'bytes': '6265'}, {'name': 'Matlab', 'bytes': '56'}, {'name': 'OCaml', 'bytes': '148372'}, {'name': 'Objective-C', 'bytes': '995127'}, {'name': 'Objective-C++', 'bytes': '436045'}, {'name': 'PHP', 'bytes': '12361'}, {'name': 'Pascal', 'bytes': '40318'}, {'name': 'Perl', 'bytes': '358808'}, {'name': 'Python', 'bytes': '60178'}, {'name': 'SAS', 'bytes': '1711'}, {'name': 'Scilab', 'bytes': '258457'}, {'name': 'Shell', 'bytes': '2610907'}, {'name': 'Tcl', 'bytes': '17983'}, {'name': 'TeX', 'bytes': '1455571'}, {'name': 'XSLT', 'bytes': '156419'}]} |
package uk.co.real_logic.aeron.common;
import java.net.InterfaceAddress;
import java.net.NetworkInterface;
import java.net.SocketException;
import java.util.Enumeration;
import java.util.List;
interface NetworkInterfaceShim
{
Enumeration<NetworkInterface> getNetworkInterfaces() throws SocketException;
List<InterfaceAddress> getInterfaceAddresses(NetworkInterface ifc);
boolean isLoopback(NetworkInterface ifc) throws SocketException;
NetworkInterfaceShim DEFAULT = new NetworkInterfaceShim()
{
public Enumeration<NetworkInterface> getNetworkInterfaces() throws SocketException
{
return NetworkInterface.getNetworkInterfaces();
}
public List<InterfaceAddress> getInterfaceAddresses(final NetworkInterface ifc)
{
return ifc.getInterfaceAddresses();
}
public boolean isLoopback(final NetworkInterface ifc) throws SocketException
{
return ifc.isLoopback();
}
};
}
| {'content_hash': '572858c544ddc9dd253a4790b6e0f3ec', 'timestamp': '', 'source': 'github', 'line_count': 33, 'max_line_length': 90, 'avg_line_length': 30.303030303030305, 'alnum_prop': 0.728, 'repo_name': 'jessefugitt/Aeron', 'id': '11a4ad5d626ba9d0d7afb8b4b986eb31982cedab', 'size': '1601', 'binary': False, 'copies': '2', 'ref': 'refs/heads/master', 'path': 'aeron-common/src/main/java/uk/co/real_logic/aeron/common/NetworkInterfaceShim.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'C++', 'bytes': '313662'}, {'name': 'CMake', 'bytes': '7737'}, {'name': 'Java', 'bytes': '990516'}, {'name': 'Shell', 'bytes': '155'}]} |
// Copyright (c) 2013 The Chromium Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "content/browser/indexed_db/leveldb/leveldb_database.h"
#include <cerrno>
#include "base/basictypes.h"
#include "base/files/file.h"
#include "base/logging.h"
#include "base/memory/scoped_ptr.h"
#include "base/metrics/histogram.h"
#include "base/strings/string16.h"
#include "base/strings/string_piece.h"
#include "base/strings/stringprintf.h"
#include "base/strings/utf_string_conversions.h"
#include "base/sys_info.h"
#include "content/browser/indexed_db/indexed_db_class_factory.h"
#include "content/browser/indexed_db/leveldb/leveldb_comparator.h"
#include "content/browser/indexed_db/leveldb/leveldb_iterator_impl.h"
#include "content/browser/indexed_db/leveldb/leveldb_write_batch.h"
#include "third_party/leveldatabase/env_chromium.h"
#include "third_party/leveldatabase/env_idb.h"
#include "third_party/leveldatabase/src/helpers/memenv/memenv.h"
#include "third_party/leveldatabase/src/include/leveldb/db.h"
#include "third_party/leveldatabase/src/include/leveldb/env.h"
#include "third_party/leveldatabase/src/include/leveldb/filter_policy.h"
#include "third_party/leveldatabase/src/include/leveldb/slice.h"
using base::StringPiece;
namespace content {
// Forcing flushes to disk at the end of a transaction guarantees that the
// data hit disk, but drastically impacts throughput when the filesystem is
// busy with background compactions. Not syncing trades off reliability for
// performance. Note that background compactions which move data from the
// log to SSTs are always done with reliable writes.
//
// Sync writes are necessary on Windows for quota calculations; POSIX
// calculates file sizes correctly even when not synced to disk.
#if defined(OS_WIN)
static const bool kSyncWrites = true;
#else
// TODO(dgrogan): Either remove the #if block or change this back to false.
// See http://crbug.com/338385.
static const bool kSyncWrites = true;
#endif
static leveldb::Slice MakeSlice(const StringPiece& s) {
return leveldb::Slice(s.begin(), s.size());
}
static StringPiece MakeStringPiece(const leveldb::Slice& s) {
return StringPiece(s.data(), s.size());
}
LevelDBDatabase::ComparatorAdapter::ComparatorAdapter(
const LevelDBComparator* comparator)
: comparator_(comparator) {}
int LevelDBDatabase::ComparatorAdapter::Compare(const leveldb::Slice& a,
const leveldb::Slice& b) const {
return comparator_->Compare(MakeStringPiece(a), MakeStringPiece(b));
}
const char* LevelDBDatabase::ComparatorAdapter::Name() const {
return comparator_->Name();
}
// TODO(jsbell): Support the methods below in the future.
void LevelDBDatabase::ComparatorAdapter::FindShortestSeparator(
std::string* start,
const leveldb::Slice& limit) const {}
void LevelDBDatabase::ComparatorAdapter::FindShortSuccessor(
std::string* key) const {}
LevelDBSnapshot::LevelDBSnapshot(LevelDBDatabase* db)
: db_(db->db_.get()), snapshot_(db_->GetSnapshot()) {}
LevelDBSnapshot::~LevelDBSnapshot() { db_->ReleaseSnapshot(snapshot_); }
LevelDBDatabase::LevelDBDatabase() {}
LevelDBDatabase::~LevelDBDatabase() {
// db_'s destructor uses comparator_adapter_; order of deletion is important.
db_.reset();
comparator_adapter_.reset();
env_.reset();
}
static leveldb::Status OpenDB(
leveldb::Comparator* comparator,
leveldb::Env* env,
const base::FilePath& path,
leveldb::DB** db,
scoped_ptr<const leveldb::FilterPolicy>* filter_policy) {
filter_policy->reset(leveldb::NewBloomFilterPolicy(10));
leveldb::Options options;
options.comparator = comparator;
options.create_if_missing = true;
options.paranoid_checks = true;
options.filter_policy = filter_policy->get();
options.reuse_logs = true;
options.compression = leveldb::kSnappyCompression;
// For info about the troubles we've run into with this parameter, see:
// https://code.google.com/p/chromium/issues/detail?id=227313#c11
options.max_open_files = 80;
options.env = env;
// ChromiumEnv assumes UTF8, converts back to FilePath before using.
leveldb::Status s = leveldb::DB::Open(options, path.AsUTF8Unsafe(), db);
return s;
}
leveldb::Status LevelDBDatabase::Destroy(const base::FilePath& file_name) {
leveldb::Options options;
options.env = leveldb::IDBEnv();
// ChromiumEnv assumes UTF8, converts back to FilePath before using.
return leveldb::DestroyDB(file_name.AsUTF8Unsafe(), options);
}
namespace {
class LockImpl : public LevelDBLock {
public:
explicit LockImpl(leveldb::Env* env, leveldb::FileLock* lock)
: env_(env), lock_(lock) {}
~LockImpl() override { env_->UnlockFile(lock_); }
private:
leveldb::Env* env_;
leveldb::FileLock* lock_;
DISALLOW_COPY_AND_ASSIGN(LockImpl);
};
} // namespace
scoped_ptr<LevelDBLock> LevelDBDatabase::LockForTesting(
const base::FilePath& file_name) {
leveldb::Env* env = leveldb::IDBEnv();
base::FilePath lock_path = file_name.AppendASCII("LOCK");
leveldb::FileLock* lock = NULL;
leveldb::Status status = env->LockFile(lock_path.AsUTF8Unsafe(), &lock);
if (!status.ok())
return scoped_ptr<LevelDBLock>();
DCHECK(lock);
return scoped_ptr<LevelDBLock>(new LockImpl(env, lock));
}
static int CheckFreeSpace(const char* const type,
const base::FilePath& file_name) {
std::string name =
std::string("WebCore.IndexedDB.LevelDB.Open") + type + "FreeDiskSpace";
int64 free_disk_space_in_k_bytes =
base::SysInfo::AmountOfFreeDiskSpace(file_name) / 1024;
if (free_disk_space_in_k_bytes < 0) {
base::Histogram::FactoryGet(
"WebCore.IndexedDB.LevelDB.FreeDiskSpaceFailure",
1,
2 /*boundary*/,
2 /*boundary*/ + 1,
base::HistogramBase::kUmaTargetedHistogramFlag)->Add(1 /*sample*/);
return -1;
}
int clamped_disk_space_k_bytes = free_disk_space_in_k_bytes > INT_MAX
? INT_MAX
: free_disk_space_in_k_bytes;
const uint64 histogram_max = static_cast<uint64>(1e9);
static_assert(histogram_max <= INT_MAX, "histogram_max too big");
base::Histogram::FactoryGet(name,
1,
histogram_max,
11 /*buckets*/,
base::HistogramBase::kUmaTargetedHistogramFlag)
->Add(clamped_disk_space_k_bytes);
return clamped_disk_space_k_bytes;
}
static void ParseAndHistogramIOErrorDetails(const std::string& histogram_name,
const leveldb::Status& s) {
leveldb_env::MethodID method;
base::File::Error error = base::File::FILE_OK;
leveldb_env::ErrorParsingResult result =
leveldb_env::ParseMethodAndError(s, &method, &error);
if (result == leveldb_env::NONE)
return;
std::string method_histogram_name(histogram_name);
method_histogram_name.append(".EnvMethod");
base::LinearHistogram::FactoryGet(
method_histogram_name,
1,
leveldb_env::kNumEntries,
leveldb_env::kNumEntries + 1,
base::HistogramBase::kUmaTargetedHistogramFlag)->Add(method);
std::string error_histogram_name(histogram_name);
if (result == leveldb_env::METHOD_AND_PFE) {
DCHECK_LT(error, 0);
error_histogram_name.append(std::string(".PFE.") +
leveldb_env::MethodIDToString(method));
base::LinearHistogram::FactoryGet(
error_histogram_name,
1,
-base::File::FILE_ERROR_MAX,
-base::File::FILE_ERROR_MAX + 1,
base::HistogramBase::kUmaTargetedHistogramFlag)->Add(-error);
}
}
static void ParseAndHistogramCorruptionDetails(
const std::string& histogram_name,
const leveldb::Status& status) {
int error = leveldb_env::GetCorruptionCode(status);
DCHECK_GE(error, 0);
std::string corruption_histogram_name(histogram_name);
corruption_histogram_name.append(".Corruption");
const int kNumPatterns = leveldb_env::GetNumCorruptionCodes();
base::LinearHistogram::FactoryGet(
corruption_histogram_name,
1,
kNumPatterns,
kNumPatterns + 1,
base::HistogramBase::kUmaTargetedHistogramFlag)->Add(error);
}
static void HistogramLevelDBError(const std::string& histogram_name,
const leveldb::Status& s) {
if (s.ok()) {
NOTREACHED();
return;
}
enum {
LEVEL_DB_NOT_FOUND,
LEVEL_DB_CORRUPTION,
LEVEL_DB_IO_ERROR,
LEVEL_DB_OTHER,
LEVEL_DB_MAX_ERROR
};
int leveldb_error = LEVEL_DB_OTHER;
if (s.IsNotFound())
leveldb_error = LEVEL_DB_NOT_FOUND;
else if (s.IsCorruption())
leveldb_error = LEVEL_DB_CORRUPTION;
else if (s.IsIOError())
leveldb_error = LEVEL_DB_IO_ERROR;
base::Histogram::FactoryGet(histogram_name,
1,
LEVEL_DB_MAX_ERROR,
LEVEL_DB_MAX_ERROR + 1,
base::HistogramBase::kUmaTargetedHistogramFlag)
->Add(leveldb_error);
if (s.IsIOError())
ParseAndHistogramIOErrorDetails(histogram_name, s);
else
ParseAndHistogramCorruptionDetails(histogram_name, s);
}
leveldb::Status LevelDBDatabase::Open(const base::FilePath& file_name,
const LevelDBComparator* comparator,
scoped_ptr<LevelDBDatabase>* result,
bool* is_disk_full) {
base::TimeTicks begin_time = base::TimeTicks::Now();
scoped_ptr<ComparatorAdapter> comparator_adapter(
new ComparatorAdapter(comparator));
leveldb::DB* db;
scoped_ptr<const leveldb::FilterPolicy> filter_policy;
const leveldb::Status s = OpenDB(comparator_adapter.get(),
leveldb::IDBEnv(),
file_name,
&db,
&filter_policy);
if (!s.ok()) {
HistogramLevelDBError("WebCore.IndexedDB.LevelDBOpenErrors", s);
int free_space_k_bytes = CheckFreeSpace("Failure", file_name);
// Disks with <100k of free space almost never succeed in opening a
// leveldb database.
if (is_disk_full)
*is_disk_full = free_space_k_bytes >= 0 && free_space_k_bytes < 100;
LOG(ERROR) << "Failed to open LevelDB database from "
<< file_name.AsUTF8Unsafe() << "," << s.ToString();
return s;
}
UMA_HISTOGRAM_MEDIUM_TIMES("WebCore.IndexedDB.LevelDB.OpenTime",
base::TimeTicks::Now() - begin_time);
CheckFreeSpace("Success", file_name);
(*result).reset(new LevelDBDatabase);
(*result)->db_ = make_scoped_ptr(db);
(*result)->comparator_adapter_ = comparator_adapter.Pass();
(*result)->comparator_ = comparator;
(*result)->filter_policy_ = filter_policy.Pass();
return s;
}
scoped_ptr<LevelDBDatabase> LevelDBDatabase::OpenInMemory(
const LevelDBComparator* comparator) {
scoped_ptr<ComparatorAdapter> comparator_adapter(
new ComparatorAdapter(comparator));
scoped_ptr<leveldb::Env> in_memory_env(leveldb::NewMemEnv(leveldb::IDBEnv()));
leveldb::DB* db;
scoped_ptr<const leveldb::FilterPolicy> filter_policy;
const leveldb::Status s = OpenDB(comparator_adapter.get(),
in_memory_env.get(),
base::FilePath(),
&db,
&filter_policy);
if (!s.ok()) {
LOG(ERROR) << "Failed to open in-memory LevelDB database: " << s.ToString();
return scoped_ptr<LevelDBDatabase>();
}
scoped_ptr<LevelDBDatabase> result(new LevelDBDatabase);
result->env_ = in_memory_env.Pass();
result->db_ = make_scoped_ptr(db);
result->comparator_adapter_ = comparator_adapter.Pass();
result->comparator_ = comparator;
result->filter_policy_ = filter_policy.Pass();
return result.Pass();
}
leveldb::Status LevelDBDatabase::Put(const StringPiece& key,
std::string* value) {
base::TimeTicks begin_time = base::TimeTicks::Now();
leveldb::WriteOptions write_options;
write_options.sync = kSyncWrites;
const leveldb::Status s =
db_->Put(write_options, MakeSlice(key), MakeSlice(*value));
if (!s.ok())
LOG(ERROR) << "LevelDB put failed: " << s.ToString();
else
UMA_HISTOGRAM_TIMES("WebCore.IndexedDB.LevelDB.PutTime",
base::TimeTicks::Now() - begin_time);
return s;
}
leveldb::Status LevelDBDatabase::Remove(const StringPiece& key) {
leveldb::WriteOptions write_options;
write_options.sync = kSyncWrites;
const leveldb::Status s = db_->Delete(write_options, MakeSlice(key));
if (!s.IsNotFound())
LOG(ERROR) << "LevelDB remove failed: " << s.ToString();
return s;
}
leveldb::Status LevelDBDatabase::Get(const StringPiece& key,
std::string* value,
bool* found,
const LevelDBSnapshot* snapshot) {
*found = false;
leveldb::ReadOptions read_options;
read_options.verify_checksums = true; // TODO(jsbell): Disable this if the
// performance impact is too great.
read_options.snapshot = snapshot ? snapshot->snapshot_ : 0;
const leveldb::Status s = db_->Get(read_options, MakeSlice(key), value);
if (s.ok()) {
*found = true;
return s;
}
if (s.IsNotFound())
return leveldb::Status::OK();
HistogramLevelDBError("WebCore.IndexedDB.LevelDBReadErrors", s);
LOG(ERROR) << "LevelDB get failed: " << s.ToString();
return s;
}
leveldb::Status LevelDBDatabase::Write(const LevelDBWriteBatch& write_batch) {
base::TimeTicks begin_time = base::TimeTicks::Now();
leveldb::WriteOptions write_options;
write_options.sync = kSyncWrites;
const leveldb::Status s =
db_->Write(write_options, write_batch.write_batch_.get());
if (!s.ok()) {
HistogramLevelDBError("WebCore.IndexedDB.LevelDBWriteErrors", s);
LOG(ERROR) << "LevelDB write failed: " << s.ToString();
} else {
UMA_HISTOGRAM_TIMES("WebCore.IndexedDB.LevelDB.WriteTime",
base::TimeTicks::Now() - begin_time);
}
return s;
}
scoped_ptr<LevelDBIterator> LevelDBDatabase::CreateIterator(
const LevelDBSnapshot* snapshot) {
leveldb::ReadOptions read_options;
read_options.verify_checksums = true; // TODO(jsbell): Disable this if the
// performance impact is too great.
read_options.snapshot = snapshot ? snapshot->snapshot_ : 0;
scoped_ptr<leveldb::Iterator> i(db_->NewIterator(read_options));
return scoped_ptr<LevelDBIterator>(
IndexedDBClassFactory::Get()->CreateIteratorImpl(i.Pass()));
}
const LevelDBComparator* LevelDBDatabase::Comparator() const {
return comparator_;
}
void LevelDBDatabase::Compact(const base::StringPiece& start,
const base::StringPiece& stop) {
const leveldb::Slice start_slice = MakeSlice(start);
const leveldb::Slice stop_slice = MakeSlice(stop);
// NULL batch means just wait for earlier writes to be done
db_->Write(leveldb::WriteOptions(), NULL);
db_->CompactRange(&start_slice, &stop_slice);
}
void LevelDBDatabase::CompactAll() { db_->CompactRange(NULL, NULL); }
} // namespace content
| {'content_hash': '4be9c7d9ff7a51482f588ca06294a5cc', 'timestamp': '', 'source': 'github', 'line_count': 429, 'max_line_length': 80, 'avg_line_length': 36.074592074592076, 'alnum_prop': 0.6566942362367537, 'repo_name': 'hefen1/chromium', 'id': 'aab1255d145a6e46d3f64e9a602365c9156d5d15', 'size': '15476', 'binary': False, 'copies': '2', 'ref': 'refs/heads/master', 'path': 'content/browser/indexed_db/leveldb/leveldb_database.cc', 'mode': '33188', 'license': 'bsd-3-clause', 'language': [{'name': 'AppleScript', 'bytes': '6973'}, {'name': 'Arduino', 'bytes': '464'}, {'name': 'Assembly', 'bytes': '23829'}, {'name': 'C', 'bytes': '4050888'}, {'name': 'C++', 'bytes': '227355953'}, {'name': 'CSS', 'bytes': '970407'}, {'name': 'HTML', 'bytes': '28896884'}, {'name': 'Java', 'bytes': '8494381'}, {'name': 'JavaScript', 'bytes': '19110753'}, {'name': 'Makefile', 'bytes': '37978'}, {'name': 'Objective-C', 'bytes': '1276474'}, {'name': 'Objective-C++', 'bytes': '7755220'}, {'name': 'PHP', 'bytes': '97817'}, {'name': 'PLpgSQL', 'bytes': '264470'}, {'name': 'Perl', 'bytes': '63937'}, {'name': 'Protocol Buffer', 'bytes': '423501'}, {'name': 'Python', 'bytes': '7622149'}, {'name': 'Shell', 'bytes': '478642'}, {'name': 'Standard ML', 'bytes': '4965'}, {'name': 'XSLT', 'bytes': '418'}, {'name': 'nesC', 'bytes': '18347'}]} |
/**
* \file
* Protects against replay attacks by comparing with the last
* unicast or broadcast frame counter of the sender.
* \author
* Konrad Krentz <[email protected]>
*/
/**
* \addtogroup llsec802154
* @{
*/
#include <net/l2_buf.h>
#include "contiki/llsec/anti-replay.h"
#include "contiki/packetbuf.h"
/* This node's current frame counter value */
static uint32_t counter;
/*---------------------------------------------------------------------------*/
void
anti_replay_set_counter(struct net_buf *buf)
{
frame802154_frame_counter_t reordered_counter;
reordered_counter.u32 = LLSEC802154_HTONL(++counter);
packetbuf_set_attr(buf, PACKETBUF_ATTR_FRAME_COUNTER_BYTES_0_1, reordered_counter.u16[0]);
packetbuf_set_attr(buf, PACKETBUF_ATTR_FRAME_COUNTER_BYTES_2_3, reordered_counter.u16[1]);
}
/*---------------------------------------------------------------------------*/
uint32_t
anti_replay_get_counter(struct net_buf *buf)
{
frame802154_frame_counter_t disordered_counter;
disordered_counter.u16[0] = packetbuf_attr(buf, PACKETBUF_ATTR_FRAME_COUNTER_BYTES_0_1);
disordered_counter.u16[1] = packetbuf_attr(buf, PACKETBUF_ATTR_FRAME_COUNTER_BYTES_2_3);
return LLSEC802154_HTONL(disordered_counter.u32);
}
/*---------------------------------------------------------------------------*/
void
anti_replay_init_info(struct net_buf *buf, struct anti_replay_info *info)
{
info->last_broadcast_counter
= info->last_unicast_counter
= anti_replay_get_counter(buf);
}
/*---------------------------------------------------------------------------*/
int
anti_replay_was_replayed(struct net_buf *buf, struct anti_replay_info *info)
{
uint32_t received_counter;
received_counter = anti_replay_get_counter(buf);
if(packetbuf_holds_broadcast(buf)) {
/* broadcast */
if(received_counter <= info->last_broadcast_counter) {
return 1;
} else {
info->last_broadcast_counter = received_counter;
return 0;
}
} else {
/* unicast */
if(received_counter <= info->last_unicast_counter) {
return 1;
} else {
info->last_unicast_counter = received_counter;
return 0;
}
}
}
/*---------------------------------------------------------------------------*/
/** @} */
| {'content_hash': '1777034a9c991d7db100787e4e62d48b', 'timestamp': '', 'source': 'github', 'line_count': 82, 'max_line_length': 92, 'avg_line_length': 28.170731707317074, 'alnum_prop': 0.561038961038961, 'repo_name': '32bitmicro/zephyr', 'id': '0db4d6b72335e7714a52d4ba29fe88b3ac52c49f', 'size': '3940', 'binary': False, 'copies': '4', 'ref': 'refs/heads/master', 'path': 'net/ip/contiki/llsec/anti-replay.c', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Assembly', 'bytes': '160164'}, {'name': 'Batchfile', 'bytes': '28019'}, {'name': 'C', 'bytes': '6173727'}, {'name': 'C++', 'bytes': '222025'}, {'name': 'Lex', 'bytes': '11196'}, {'name': 'Makefile', 'bytes': '132295'}, {'name': 'Objective-C', 'bytes': '1912'}, {'name': 'Perl', 'bytes': '213268'}, {'name': 'Python', 'bytes': '109645'}, {'name': 'Shell', 'bytes': '44817'}, {'name': 'Yacc', 'bytes': '15396'}]} |
package com.acework.js.components.bootstrap
import com.acework.js.utils.{Mappable, Mergeable}
import japgolly.scalajs.react._
import japgolly.scalajs.react.vdom.prefix_<^._
import scala.scalajs.js.{UndefOr, undefined}
/**
* Created by weiyin on 10/03/15.
*/
object Well extends BootstrapComponent {
override type P = Well
override type S = Unit
override type B = Unit
override type N = TopNode
override def defaultProps = Well()
case class Well(bsClass: UndefOr[Classes.Value] = Classes.well,
bsStyle: UndefOr[Styles.Value] = undefined,
bsSize: UndefOr[Sizes.Value] = undefined,
addClasses: String = "")
extends BsProps with MergeableProps[Well] {
def merge(t: Map[String, Any]): Well = implicitly[Mergeable[Well]].merge(this, t)
def asMap: Map[String, Any] = implicitly[Mappable[Well]].toMap(this)
def apply(children: ReactNode*) = component(this, children)
def apply() = component(this)
}
override val component = ReactComponentB[Well]("Well")
.render { (P, C) =>
// TODO spread props
<.div(^.classSet1M(P.addClasses, P.bsClassSet))(C)
}.build
}
| {'content_hash': '2c0f045ddc884f768217779e99f20d1a', 'timestamp': '', 'source': 'github', 'line_count': 43, 'max_line_length': 85, 'avg_line_length': 27.13953488372093, 'alnum_prop': 0.6709511568123393, 'repo_name': 'weiyinteo/scalajs-react-bootstrap', 'id': '6db3f50e028d4f9b8030d93ff00d1a9a724bfc3b', 'size': '1167', 'binary': False, 'copies': '2', 'ref': 'refs/heads/master', 'path': 'core/src/main/scala/com/acework/js/components/bootstrap/Well.scala', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '6518'}, {'name': 'HTML', 'bytes': '1421'}, {'name': 'Scala', 'bytes': '334544'}]} |
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>edu.clemson</groupId>
<artifactId>resolve-master</artifactId>
<version>0.0.1-SNAPSHOT</version>
<relativePath>../pom.xml</relativePath>
</parent>
<artifactId>resolve-runtime</artifactId>
<name>RESOLVE Runtime</name>
<description>The RESOLVE Runtime</description>
<build>
<sourceDirectory>src</sourceDirectory>
<plugins>
</plugins>
</build>
</project> | {'content_hash': '9db3f2bd5c8c66d2148862f9b7c04f00', 'timestamp': '', 'source': 'github', 'line_count': 19, 'max_line_length': 201, 'avg_line_length': 36.578947368421055, 'alnum_prop': 0.6589928057553956, 'repo_name': 'Welchd1/resolve-lite', 'id': '39f4ca5c089d56d93766a7e02d51bc9da14cd42e', 'size': '695', 'binary': False, 'copies': '3', 'ref': 'refs/heads/master', 'path': 'runtime/pom.xml', 'mode': '33188', 'license': 'bsd-3-clause', 'language': [{'name': 'ANTLR', 'bytes': '15010'}, {'name': 'Java', 'bytes': '717515'}]} |
package adf.launcher.option;
import adf.launcher.ConfigKey;
import rescuecore2.config.Config;
public class OptionPoliceOffice extends Option
{
@Override
public String getKey()
{
return "-po";
}
@Override
public void setValue(Config config, String[] datas)
{
if(datas.length == 2)
{
config.setValue(ConfigKey.KEY_POLICE_OFFICE_COUNT, datas[1]);
}
}
} | {'content_hash': '24f95fa27e0fccff88aa1574153e833d', 'timestamp': '', 'source': 'github', 'line_count': 23, 'max_line_length': 64, 'avg_line_length': 16.17391304347826, 'alnum_prop': 0.717741935483871, 'repo_name': 'tkmnet/RCRS-ADF', 'id': 'cc8bf5d4ac94dcea0fe5311e0208eaa98c61bf98', 'size': '372', 'binary': False, 'copies': '2', 'ref': 'refs/heads/master', 'path': 'modules/core/src/main/java/adf/launcher/option/OptionPoliceOffice.java', 'mode': '33188', 'license': 'bsd-2-clause', 'language': [{'name': 'Assembly', 'bytes': '277'}, {'name': 'Batchfile', 'bytes': '2394'}, {'name': 'C', 'bytes': '115742'}, {'name': 'C++', 'bytes': '4876'}, {'name': 'CSS', 'bytes': '104604'}, {'name': 'GAP', 'bytes': '176'}, {'name': 'Groovy', 'bytes': '1142351'}, {'name': 'HTML', 'bytes': '28618885'}, {'name': 'Java', 'bytes': '10250957'}, {'name': 'JavaScript', 'bytes': '190908'}, {'name': 'Objective-C', 'bytes': '698'}, {'name': 'Objective-C++', 'bytes': '442'}, {'name': 'Scala', 'bytes': '3073'}, {'name': 'Shell', 'bytes': '16330'}]} |
#ifndef _SET_RADAR_LOD_ACTION_H_
#define _SET_RADAR_LOD_ACTION_H_
#ifndef _ACTION_H_
#include "Action.h"
#endif// _ACTION_H_
namespace Training
{
//------------------------------------------------------------------------------
// class definitions
//------------------------------------------------------------------------------
class SetRadarLODAction : public Action
{
public:
/* void */ SetRadarLODAction (RadarImage::RadarLOD radarLOD);
virtual /* void */ ~SetRadarLODAction (void);
virtual void Execute (void);
protected:
RadarImage::RadarLOD m_radarLOD;
};
//------------------------------------------------------------------------------
}
#endif //_SET_RADAR_LOD_ACTION_H_
| {'content_hash': '5d58036fec80b4bd6c5b57769ea7360e', 'timestamp': '', 'source': 'github', 'line_count': 28, 'max_line_length': 90, 'avg_line_length': 29.678571428571427, 'alnum_prop': 0.3850782190132371, 'repo_name': 'AllegianceZone/Allegiance', 'id': '4431344ef9dd4614b2cf5ba28fb5240e002ccb7b', 'size': '1065', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/training/SetRadarLODAction.h', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Assembly', 'bytes': '26927'}, {'name': 'Batchfile', 'bytes': '20387'}, {'name': 'C', 'bytes': '3213698'}, {'name': 'C++', 'bytes': '11383849'}, {'name': 'CSS', 'bytes': '1905'}, {'name': 'HTML', 'bytes': '369500'}, {'name': 'JavaScript', 'bytes': '125561'}, {'name': 'Makefile', 'bytes': '9519'}, {'name': 'Objective-C', 'bytes': '41562'}, {'name': 'Perl', 'bytes': '3074'}, {'name': 'PigLatin', 'bytes': '250645'}, {'name': 'Roff', 'bytes': '5275'}, {'name': 'Visual Basic', 'bytes': '7253'}, {'name': 'XSLT', 'bytes': '19495'}]} |
package azure
import (
"crypto/rsa"
"crypto/x509"
"fmt"
"io"
"io/ioutil"
"time"
"k8s.io/client-go/util/flowcontrol"
"k8s.io/kubernetes/pkg/cloudprovider"
"k8s.io/kubernetes/pkg/controller"
"k8s.io/kubernetes/pkg/version"
"github.com/Azure/azure-sdk-for-go/arm/compute"
"github.com/Azure/azure-sdk-for-go/arm/disk"
"github.com/Azure/azure-sdk-for-go/arm/network"
"github.com/Azure/azure-sdk-for-go/arm/storage"
"github.com/Azure/go-autorest/autorest"
"github.com/Azure/go-autorest/autorest/adal"
"github.com/Azure/go-autorest/autorest/azure"
"github.com/ghodss/yaml"
"github.com/golang/glog"
"golang.org/x/crypto/pkcs12"
"k8s.io/apimachinery/pkg/util/wait"
)
const (
// CloudProviderName is the value used for the --cloud-provider flag
CloudProviderName = "azure"
rateLimitQPSDefault = 1.0
rateLimitBucketDefault = 5
backoffRetriesDefault = 6
backoffExponentDefault = 1.5
backoffDurationDefault = 5 // in seconds
backoffJitterDefault = 1.0
)
// Config holds the configuration parsed from the --cloud-config flag
// All fields are required unless otherwise specified
type Config struct {
// The cloud environment identifier. Takes values from https://github.com/Azure/go-autorest/blob/ec5f4903f77ed9927ac95b19ab8e44ada64c1356/autorest/azure/environments.go#L13
Cloud string `json:"cloud" yaml:"cloud"`
// The AAD Tenant ID for the Subscription that the cluster is deployed in
TenantID string `json:"tenantId" yaml:"tenantId"`
// The ID of the Azure Subscription that the cluster is deployed in
SubscriptionID string `json:"subscriptionId" yaml:"subscriptionId"`
// The name of the resource group that the cluster is deployed in
ResourceGroup string `json:"resourceGroup" yaml:"resourceGroup"`
// The location of the resource group that the cluster is deployed in
Location string `json:"location" yaml:"location"`
// The name of the VNet that the cluster is deployed in
VnetName string `json:"vnetName" yaml:"vnetName"`
// The name of the resource group that the Vnet is deployed in
VnetResourceGroup string `json:"vnetResourceGroup" yaml:"vnetResourceGroup"`
// The name of the subnet that the cluster is deployed in
SubnetName string `json:"subnetName" yaml:"subnetName"`
// The name of the security group attached to the cluster's subnet
SecurityGroupName string `json:"securityGroupName" yaml:"securityGroupName"`
// (Optional in 1.6) The name of the route table attached to the subnet that the cluster is deployed in
RouteTableName string `json:"routeTableName" yaml:"routeTableName"`
// (Optional) The name of the availability set that should be used as the load balancer backend
// If this is set, the Azure cloudprovider will only add nodes from that availability set to the load
// balancer backend pool. If this is not set, and multiple agent pools (availability sets) are used, then
// the cloudprovider will try to add all nodes to a single backend pool which is forbidden.
// In other words, if you use multiple agent pools (availability sets), you MUST set this field.
PrimaryAvailabilitySetName string `json:"primaryAvailabilitySetName" yaml:"primaryAvailabilitySetName"`
// The ClientID for an AAD application with RBAC access to talk to Azure RM APIs
AADClientID string `json:"aadClientId" yaml:"aadClientId"`
// The ClientSecret for an AAD application with RBAC access to talk to Azure RM APIs
AADClientSecret string `json:"aadClientSecret" yaml:"aadClientSecret"`
// The path of a client certificate for an AAD application with RBAC access to talk to Azure RM APIs
AADClientCertPath string `json:"aadClientCertPath" yaml:"aadClientCertPath"`
// The password of the client certificate for an AAD application with RBAC access to talk to Azure RM APIs
AADClientCertPassword string `json:"aadClientCertPassword" yaml:"aadClientCertPassword"`
// Enable exponential backoff to manage resource request retries
CloudProviderBackoff bool `json:"cloudProviderBackoff" yaml:"cloudProviderBackoff"`
// Backoff retry limit
CloudProviderBackoffRetries int `json:"cloudProviderBackoffRetries" yaml:"cloudProviderBackoffRetries"`
// Backoff exponent
CloudProviderBackoffExponent float64 `json:"cloudProviderBackoffExponent" yaml:"cloudProviderBackoffExponent"`
// Backoff duration
CloudProviderBackoffDuration int `json:"cloudProviderBackoffDuration" yaml:"cloudProviderBackoffDuration"`
// Backoff jitter
CloudProviderBackoffJitter float64 `json:"cloudProviderBackoffJitter" yaml:"cloudProviderBackoffJitter"`
// Enable rate limiting
CloudProviderRateLimit bool `json:"cloudProviderRateLimit" yaml:"cloudProviderRateLimit"`
// Rate limit QPS
CloudProviderRateLimitQPS float32 `json:"cloudProviderRateLimitQPS" yaml:"cloudProviderRateLimitQPS"`
// Rate limit Bucket Size
CloudProviderRateLimitBucket int `json:"cloudProviderRateLimitBucket" yaml:"cloudProviderRateLimitBucket"`
// Use instance metadata service where possible
UseInstanceMetadata bool `json:"useInstanceMetadata" yaml:"useInstanceMetadata"`
// Use managed service identity for the virtual machine to access Azure ARM APIs
UseManagedIdentityExtension bool `json:"useManagedIdentityExtension"`
}
// Cloud holds the config and clients
type Cloud struct {
Config
Environment azure.Environment
RoutesClient network.RoutesClient
SubnetsClient network.SubnetsClient
InterfacesClient network.InterfacesClient
RouteTablesClient network.RouteTablesClient
LoadBalancerClient network.LoadBalancersClient
PublicIPAddressesClient network.PublicIPAddressesClient
SecurityGroupsClient network.SecurityGroupsClient
VirtualMachinesClient compute.VirtualMachinesClient
StorageAccountClient storage.AccountsClient
DisksClient disk.DisksClient
operationPollRateLimiter flowcontrol.RateLimiter
resourceRequestBackoff wait.Backoff
metadata *InstanceMetadata
*BlobDiskController
*ManagedDiskController
*controllerCommon
}
func init() {
cloudprovider.RegisterCloudProvider(CloudProviderName, NewCloud)
}
// decodePkcs12 decodes a PKCS#12 client certificate by extracting the public certificate and
// the private RSA key
func decodePkcs12(pkcs []byte, password string) (*x509.Certificate, *rsa.PrivateKey, error) {
privateKey, certificate, err := pkcs12.Decode(pkcs, password)
if err != nil {
return nil, nil, fmt.Errorf("decoding the PKCS#12 client certificate: %v", err)
}
rsaPrivateKey, isRsaKey := privateKey.(*rsa.PrivateKey)
if !isRsaKey {
return nil, nil, fmt.Errorf("PKCS#12 certificate must contain a RSA private key")
}
return certificate, rsaPrivateKey, nil
}
// GetServicePrincipalToken creates a new service principal token based on the configuration
func GetServicePrincipalToken(config *Config, env *azure.Environment) (*adal.ServicePrincipalToken, error) {
oauthConfig, err := adal.NewOAuthConfig(env.ActiveDirectoryEndpoint, config.TenantID)
if err != nil {
return nil, fmt.Errorf("creating the OAuth config: %v", err)
}
if config.UseManagedIdentityExtension {
glog.V(2).Infoln("azure: using managed identity extension to retrieve access token")
msiEndpoint, err := adal.GetMSIVMEndpoint()
if err != nil {
return nil, fmt.Errorf("Getting the managed service identity endpoint: %v", err)
}
return adal.NewServicePrincipalTokenFromMSI(
msiEndpoint,
env.ServiceManagementEndpoint)
}
if len(config.AADClientSecret) > 0 {
glog.V(2).Infoln("azure: using client_id+client_secret to retrieve access token")
return adal.NewServicePrincipalToken(
*oauthConfig,
config.AADClientID,
config.AADClientSecret,
env.ServiceManagementEndpoint)
}
if len(config.AADClientCertPath) > 0 && len(config.AADClientCertPassword) > 0 {
glog.V(2).Infoln("azure: using jwt client_assertion (client_cert+client_private_key) to retrieve access token")
certData, err := ioutil.ReadFile(config.AADClientCertPath)
if err != nil {
return nil, fmt.Errorf("reading the client certificate from file %s: %v", config.AADClientCertPath, err)
}
certificate, privateKey, err := decodePkcs12(certData, config.AADClientCertPassword)
if err != nil {
return nil, fmt.Errorf("decoding the client certificate: %v", err)
}
return adal.NewServicePrincipalTokenFromCertificate(
*oauthConfig,
config.AADClientID,
certificate,
privateKey,
env.ServiceManagementEndpoint)
}
return nil, fmt.Errorf("No credentials provided for AAD application %s", config.AADClientID)
}
// NewCloud returns a Cloud with initialized clients
func NewCloud(configReader io.Reader) (cloudprovider.Interface, error) {
config, env, err := ParseConfig(configReader)
if err != nil {
return nil, err
}
az := Cloud{
Config: *config,
Environment: *env,
}
servicePrincipalToken, err := GetServicePrincipalToken(config, env)
if err != nil {
return nil, err
}
az.SubnetsClient = network.NewSubnetsClient(az.SubscriptionID)
az.SubnetsClient.BaseURI = az.Environment.ResourceManagerEndpoint
az.SubnetsClient.Authorizer = autorest.NewBearerAuthorizer(servicePrincipalToken)
az.SubnetsClient.PollingDelay = 5 * time.Second
configureUserAgent(&az.SubnetsClient.Client)
az.RouteTablesClient = network.NewRouteTablesClient(az.SubscriptionID)
az.RouteTablesClient.BaseURI = az.Environment.ResourceManagerEndpoint
az.RouteTablesClient.Authorizer = autorest.NewBearerAuthorizer(servicePrincipalToken)
az.RouteTablesClient.PollingDelay = 5 * time.Second
configureUserAgent(&az.RouteTablesClient.Client)
az.RoutesClient = network.NewRoutesClient(az.SubscriptionID)
az.RoutesClient.BaseURI = az.Environment.ResourceManagerEndpoint
az.RoutesClient.Authorizer = autorest.NewBearerAuthorizer(servicePrincipalToken)
az.RoutesClient.PollingDelay = 5 * time.Second
configureUserAgent(&az.RoutesClient.Client)
az.InterfacesClient = network.NewInterfacesClient(az.SubscriptionID)
az.InterfacesClient.BaseURI = az.Environment.ResourceManagerEndpoint
az.InterfacesClient.Authorizer = autorest.NewBearerAuthorizer(servicePrincipalToken)
az.InterfacesClient.PollingDelay = 5 * time.Second
configureUserAgent(&az.InterfacesClient.Client)
az.LoadBalancerClient = network.NewLoadBalancersClient(az.SubscriptionID)
az.LoadBalancerClient.BaseURI = az.Environment.ResourceManagerEndpoint
az.LoadBalancerClient.Authorizer = autorest.NewBearerAuthorizer(servicePrincipalToken)
az.LoadBalancerClient.PollingDelay = 5 * time.Second
configureUserAgent(&az.LoadBalancerClient.Client)
az.VirtualMachinesClient = compute.NewVirtualMachinesClient(az.SubscriptionID)
az.VirtualMachinesClient.BaseURI = az.Environment.ResourceManagerEndpoint
az.VirtualMachinesClient.Authorizer = autorest.NewBearerAuthorizer(servicePrincipalToken)
az.VirtualMachinesClient.PollingDelay = 5 * time.Second
configureUserAgent(&az.VirtualMachinesClient.Client)
az.PublicIPAddressesClient = network.NewPublicIPAddressesClient(az.SubscriptionID)
az.PublicIPAddressesClient.BaseURI = az.Environment.ResourceManagerEndpoint
az.PublicIPAddressesClient.Authorizer = autorest.NewBearerAuthorizer(servicePrincipalToken)
az.PublicIPAddressesClient.PollingDelay = 5 * time.Second
configureUserAgent(&az.PublicIPAddressesClient.Client)
az.SecurityGroupsClient = network.NewSecurityGroupsClient(az.SubscriptionID)
az.SecurityGroupsClient.BaseURI = az.Environment.ResourceManagerEndpoint
az.SecurityGroupsClient.Authorizer = autorest.NewBearerAuthorizer(servicePrincipalToken)
az.SecurityGroupsClient.PollingDelay = 5 * time.Second
configureUserAgent(&az.SecurityGroupsClient.Client)
az.StorageAccountClient = storage.NewAccountsClientWithBaseURI(az.Environment.ResourceManagerEndpoint, az.SubscriptionID)
az.StorageAccountClient.Authorizer = autorest.NewBearerAuthorizer(servicePrincipalToken)
configureUserAgent(&az.StorageAccountClient.Client)
az.DisksClient = disk.NewDisksClientWithBaseURI(az.Environment.ResourceManagerEndpoint, az.SubscriptionID)
az.DisksClient.Authorizer = autorest.NewBearerAuthorizer(servicePrincipalToken)
configureUserAgent(&az.DisksClient.Client)
// Conditionally configure rate limits
if az.CloudProviderRateLimit {
// Assign rate limit defaults if no configuration was passed in
if az.CloudProviderRateLimitQPS == 0 {
az.CloudProviderRateLimitQPS = rateLimitQPSDefault
}
if az.CloudProviderRateLimitBucket == 0 {
az.CloudProviderRateLimitBucket = rateLimitBucketDefault
}
az.operationPollRateLimiter = flowcontrol.NewTokenBucketRateLimiter(
az.CloudProviderRateLimitQPS,
az.CloudProviderRateLimitBucket)
glog.V(2).Infof("Azure cloudprovider using rate limit config: QPS=%g, bucket=%d",
az.CloudProviderRateLimitQPS,
az.CloudProviderRateLimitBucket)
} else {
// if rate limits are configured off, az.operationPollRateLimiter.Accept() is a no-op
az.operationPollRateLimiter = flowcontrol.NewFakeAlwaysRateLimiter()
}
// Conditionally configure resource request backoff
if az.CloudProviderBackoff {
// Assign backoff defaults if no configuration was passed in
if az.CloudProviderBackoffRetries == 0 {
az.CloudProviderBackoffRetries = backoffRetriesDefault
}
if az.CloudProviderBackoffExponent == 0 {
az.CloudProviderBackoffExponent = backoffExponentDefault
}
if az.CloudProviderBackoffDuration == 0 {
az.CloudProviderBackoffDuration = backoffDurationDefault
}
if az.CloudProviderBackoffJitter == 0 {
az.CloudProviderBackoffJitter = backoffJitterDefault
}
az.resourceRequestBackoff = wait.Backoff{
Steps: az.CloudProviderBackoffRetries,
Factor: az.CloudProviderBackoffExponent,
Duration: time.Duration(az.CloudProviderBackoffDuration) * time.Second,
Jitter: az.CloudProviderBackoffJitter,
}
glog.V(2).Infof("Azure cloudprovider using retry backoff: retries=%d, exponent=%f, duration=%d, jitter=%f",
az.CloudProviderBackoffRetries,
az.CloudProviderBackoffExponent,
az.CloudProviderBackoffDuration,
az.CloudProviderBackoffJitter)
}
az.metadata = NewInstanceMetadata()
if err := initDiskControllers(&az); err != nil {
return nil, err
}
return &az, nil
}
// ParseConfig returns a parsed configuration and azure.Environment for an Azure cloudprovider config file
func ParseConfig(configReader io.Reader) (*Config, *azure.Environment, error) {
var config Config
var env azure.Environment
if configReader == nil {
return &config, &env, nil
}
configContents, err := ioutil.ReadAll(configReader)
if err != nil {
return nil, nil, err
}
err = yaml.Unmarshal(configContents, &config)
if err != nil {
return nil, nil, err
}
if config.Cloud == "" {
env = azure.PublicCloud
} else {
env, err = azure.EnvironmentFromName(config.Cloud)
if err != nil {
return nil, nil, err
}
}
return &config, &env, nil
}
// Initialize passes a Kubernetes clientBuilder interface to the cloud provider
func (az *Cloud) Initialize(clientBuilder controller.ControllerClientBuilder) {}
// LoadBalancer returns a balancer interface. Also returns true if the interface is supported, false otherwise.
func (az *Cloud) LoadBalancer() (cloudprovider.LoadBalancer, bool) {
return az, true
}
// Instances returns an instances interface. Also returns true if the interface is supported, false otherwise.
func (az *Cloud) Instances() (cloudprovider.Instances, bool) {
return az, true
}
// Zones returns a zones interface. Also returns true if the interface is supported, false otherwise.
func (az *Cloud) Zones() (cloudprovider.Zones, bool) {
return az, true
}
// Clusters returns a clusters interface. Also returns true if the interface is supported, false otherwise.
func (az *Cloud) Clusters() (cloudprovider.Clusters, bool) {
return nil, false
}
// Routes returns a routes interface along with whether the interface is supported.
func (az *Cloud) Routes() (cloudprovider.Routes, bool) {
return az, true
}
// ScrubDNS provides an opportunity for cloud-provider-specific code to process DNS settings for pods.
func (az *Cloud) ScrubDNS(nameservers, searches []string) (nsOut, srchOut []string) {
return nameservers, searches
}
// HasClusterID returns true if the cluster has a clusterID
func (az *Cloud) HasClusterID() bool {
return true
}
// ProviderName returns the cloud provider ID.
func (az *Cloud) ProviderName() string {
return CloudProviderName
}
// configureUserAgent configures the autorest client with a user agent that
// includes "kubernetes" and the full kubernetes git version string
// example:
// Azure-SDK-for-Go/7.0.1-beta arm-network/2016-09-01; kubernetes-cloudprovider/v1.7.0-alpha.2.711+a2fadef8170bb0-dirty;
func configureUserAgent(client *autorest.Client) {
k8sVersion := version.Get().GitVersion
client.UserAgent = fmt.Sprintf("%s; kubernetes-cloudprovider/%s", client.UserAgent, k8sVersion)
}
func initDiskControllers(az *Cloud) error {
// Common controller contains the function
// needed by both blob disk and managed disk controllers
common := &controllerCommon{
aadResourceEndPoint: az.Environment.ServiceManagementEndpoint,
clientID: az.AADClientID,
clientSecret: az.AADClientSecret,
location: az.Location,
storageEndpointSuffix: az.Environment.StorageEndpointSuffix,
managementEndpoint: az.Environment.ResourceManagerEndpoint,
resourceGroup: az.ResourceGroup,
tenantID: az.TenantID,
tokenEndPoint: az.Environment.ActiveDirectoryEndpoint,
subscriptionID: az.SubscriptionID,
cloud: az,
}
// BlobDiskController: contains the function needed to
// create/attach/detach/delete blob based (unmanaged disks)
blobController, err := newBlobDiskController(common)
if err != nil {
return fmt.Errorf("AzureDisk - failed to init Blob Disk Controller with error (%s)", err.Error())
}
// ManagedDiskController: contains the functions needed to
// create/attach/detach/delete managed disks
managedController, err := newManagedDiskController(common)
if err != nil {
return fmt.Errorf("AzureDisk - failed to init Managed Disk Controller with error (%s)", err.Error())
}
az.BlobDiskController = blobController
az.ManagedDiskController = managedController
az.controllerCommon = common
return nil
}
| {'content_hash': 'ddceafad59fd4554816645c7d8d72191', 'timestamp': '', 'source': 'github', 'line_count': 440, 'max_line_length': 173, 'avg_line_length': 41.402272727272724, 'alnum_prop': 0.7819070099357742, 'repo_name': 'guangxuli/kubernetes', 'id': 'ee9ebf352fae9752d3970f87599c2284327e7c59', 'size': '18786', 'binary': False, 'copies': '4', 'ref': 'refs/heads/master', 'path': 'pkg/cloudprovider/providers/azure/azure.go', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'C', 'bytes': '2525'}, {'name': 'Go', 'bytes': '37484418'}, {'name': 'HTML', 'bytes': '1199467'}, {'name': 'Makefile', 'bytes': '73377'}, {'name': 'PowerShell', 'bytes': '4261'}, {'name': 'Python', 'bytes': '2546728'}, {'name': 'Ruby', 'bytes': '1733'}, {'name': 'SaltStack', 'bytes': '52094'}, {'name': 'Shell', 'bytes': '1642811'}]} |
module API
end
| {'content_hash': '2c708cc6e49eed2c0bf9a5a6704eb0b9', 'timestamp': '', 'source': 'github', 'line_count': 2, 'max_line_length': 10, 'avg_line_length': 7.5, 'alnum_prop': 0.8, 'repo_name': 'metova/metova-rails', 'id': '6e6f9edc6c2e514e6541b35d6b4cff91c29bb9e5', 'size': '15', 'binary': False, 'copies': '2', 'ref': 'refs/heads/master', 'path': 'lib/metova/api.rb', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '1500'}, {'name': 'HTML', 'bytes': '5647'}, {'name': 'JavaScript', 'bytes': '1351'}, {'name': 'Ruby', 'bytes': '87018'}]} |
<?php
/** Zend_Config */
require_once 'Zend/Config.php';
/**
* @package Zend_Controller
* @subpackage Router
* @copyright Copyright (c) 2005-2008 Zend Technologies USA Inc. (http://www.zend.com)
* @license http://framework.zend.com/license/new-bsd New BSD License
*/
interface Zend_Controller_Router_Route_Interface {
public function match($path);
public function assemble($data = array(), $reset = false, $encode = false);
public static function getInstance(Zend_Config $config);
}
| {'content_hash': '10413756cf50b3bc54f82fa578debc52', 'timestamp': '', 'source': 'github', 'line_count': 18, 'max_line_length': 87, 'avg_line_length': 29.61111111111111, 'alnum_prop': 0.6660412757973734, 'repo_name': 'ankuradhey/dealtrip', 'id': 'd20be20338c6e3891acaee16883b5edaf245e17d', 'size': '1299', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'library/Zend/Controller/Router/Route/Interface.php', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'ApacheConf', 'bytes': '395'}, {'name': 'CSS', 'bytes': '674987'}, {'name': 'HTML', 'bytes': '2367754'}, {'name': 'JavaScript', 'bytes': '2191952'}, {'name': 'PHP', 'bytes': '16297944'}, {'name': 'Python', 'bytes': '42582'}]} |
namespace bluez {
// This class contains a Bluetooth service attribute. A service attribute is
// defined by the following fields,
// type: This is the type of the attribute. Along with being any of the
// fixed types, an attribute can also be of type sequence, which means
// that it contains an array of other attributes.
// size: This is the size of the attribute. This can be variable for each type.
// For example, a UUID can have the sizes, 2, 4 or 16 bytes.
// value: This is the raw value of the attribute. For example, for a UUID, it
// will be the string representation of the UUID. For a sequence, it
// will be an array of other attributes.
class DEVICE_BLUETOOTH_EXPORT BluetoothServiceAttributeValueBlueZ {
public:
enum Type { NULLTYPE = 0, UINT, INT, UUID, STRING, BOOL, SEQUENCE, URL };
using Sequence = std::vector<BluetoothServiceAttributeValueBlueZ>;
BluetoothServiceAttributeValueBlueZ();
BluetoothServiceAttributeValueBlueZ(Type type,
size_t size,
std::unique_ptr<base::Value> value);
explicit BluetoothServiceAttributeValueBlueZ(
std::unique_ptr<Sequence> sequence);
BluetoothServiceAttributeValueBlueZ(
const BluetoothServiceAttributeValueBlueZ& attribute);
BluetoothServiceAttributeValueBlueZ& operator=(
const BluetoothServiceAttributeValueBlueZ& attribute);
~BluetoothServiceAttributeValueBlueZ();
Type type() const { return type_; }
size_t size() const { return size_; }
const Sequence& sequence() const { return *sequence_.get(); }
const base::Value& value() const { return *value_.get(); }
private:
Type type_;
size_t size_;
std::unique_ptr<base::Value> value_;
std::unique_ptr<Sequence> sequence_;
};
} // namespace bluez
#endif // DEVICE_BLUETOOTH_BLUEZ_BLUETOOTH_SERVICE_ATTRIBUTE_VALUE_BLUEZ_H_
| {'content_hash': '6ddee5f2d41f0e4baba9525148d757df', 'timestamp': '', 'source': 'github', 'line_count': 45, 'max_line_length': 80, 'avg_line_length': 42.266666666666666, 'alnum_prop': 0.7018927444794952, 'repo_name': 'ssaroha/node-webrtc', 'id': 'fdd291a5f100e4cdced1af2460235afdcf30b4ec', 'size': '2346', 'binary': False, 'copies': '14', 'ref': 'refs/heads/develop', 'path': 'third_party/webrtc/include/chromium/src/device/bluetooth/bluez/bluetooth_service_attribute_value_bluez.h', 'mode': '33188', 'license': 'bsd-2-clause', 'language': [{'name': 'Batchfile', 'bytes': '6179'}, {'name': 'C', 'bytes': '2679'}, {'name': 'C++', 'bytes': '54327'}, {'name': 'HTML', 'bytes': '434'}, {'name': 'JavaScript', 'bytes': '42707'}, {'name': 'Python', 'bytes': '3835'}]} |
use ::{BroadcastMode, Instruction, MaskReg, MergeMode, Mnemonic, OperandSize, Reg, RoundingMode};
use ::RegType::*;
use ::instruction_def::*;
use ::Operand::*;
use ::Reg::*;
use ::RegScale::*;
use ::test::run_test;
#[test]
fn vmovupd_1() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(Direct(XMM6)), operand2: Some(Direct(XMM1)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: None, sae: false, mask: None, broadcast: None }, &[197, 249, 16, 241], OperandSize::Dword)
}
#[test]
fn vmovupd_2() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(Direct(XMM0)), operand2: Some(IndirectScaledIndexed(ECX, EDX, Four, Some(OperandSize::Xmmword), None)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: None, sae: false, mask: None, broadcast: None }, &[197, 249, 16, 4, 145], OperandSize::Dword)
}
#[test]
fn vmovupd_3() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(Direct(XMM3)), operand2: Some(Direct(XMM5)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: None, sae: false, mask: None, broadcast: None }, &[197, 249, 16, 221], OperandSize::Qword)
}
#[test]
fn vmovupd_4() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(Direct(XMM3)), operand2: Some(Indirect(RAX, Some(OperandSize::Xmmword), None)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: None, sae: false, mask: None, broadcast: None }, &[197, 249, 16, 24], OperandSize::Qword)
}
#[test]
fn vmovupd_5() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(Direct(YMM0)), operand2: Some(Direct(YMM6)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: None, sae: false, mask: None, broadcast: None }, &[197, 253, 16, 198], OperandSize::Dword)
}
#[test]
fn vmovupd_6() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(Direct(YMM5)), operand2: Some(Indirect(ESI, Some(OperandSize::Ymmword), None)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: None, sae: false, mask: None, broadcast: None }, &[197, 253, 16, 46], OperandSize::Dword)
}
#[test]
fn vmovupd_7() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(Direct(YMM5)), operand2: Some(Direct(YMM2)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: None, sae: false, mask: None, broadcast: None }, &[197, 253, 16, 234], OperandSize::Qword)
}
#[test]
fn vmovupd_8() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(Direct(YMM2)), operand2: Some(Indirect(RSI, Some(OperandSize::Ymmword), None)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: None, sae: false, mask: None, broadcast: None }, &[197, 253, 16, 22], OperandSize::Qword)
}
#[test]
fn vmovupd_9() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(Direct(XMM6)), operand2: Some(Direct(XMM7)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: Some(MergeMode::Zero), sae: false, mask: Some(MaskReg::K4), broadcast: None }, &[98, 241, 253, 140, 16, 247], OperandSize::Dword)
}
#[test]
fn vmovupd_10() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(Direct(XMM1)), operand2: Some(IndirectScaledIndexed(EDX, ECX, Four, Some(OperandSize::Xmmword), None)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: Some(MergeMode::Zero), sae: false, mask: Some(MaskReg::K1), broadcast: None }, &[98, 241, 253, 137, 16, 12, 138], OperandSize::Dword)
}
#[test]
fn vmovupd_11() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(Direct(XMM20)), operand2: Some(Direct(XMM4)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: Some(MergeMode::Zero), sae: false, mask: Some(MaskReg::K6), broadcast: None }, &[98, 225, 253, 142, 16, 228], OperandSize::Qword)
}
#[test]
fn vmovupd_12() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(Direct(XMM17)), operand2: Some(IndirectDisplaced(RCX, 1305176396, Some(OperandSize::Xmmword), None)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: Some(MergeMode::Zero), sae: false, mask: Some(MaskReg::K1), broadcast: None }, &[98, 225, 253, 137, 16, 137, 76, 105, 203, 77], OperandSize::Qword)
}
#[test]
fn vmovupd_13() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(Direct(YMM7)), operand2: Some(Direct(YMM4)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: Some(MergeMode::Zero), sae: false, mask: Some(MaskReg::K7), broadcast: None }, &[98, 241, 253, 175, 16, 252], OperandSize::Dword)
}
#[test]
fn vmovupd_14() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(Direct(YMM5)), operand2: Some(Indirect(EAX, Some(OperandSize::Ymmword), None)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: Some(MergeMode::Zero), sae: false, mask: Some(MaskReg::K2), broadcast: None }, &[98, 241, 253, 170, 16, 40], OperandSize::Dword)
}
#[test]
fn vmovupd_15() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(Direct(YMM28)), operand2: Some(Direct(YMM30)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: Some(MergeMode::Zero), sae: false, mask: Some(MaskReg::K5), broadcast: None }, &[98, 1, 253, 173, 16, 230], OperandSize::Qword)
}
#[test]
fn vmovupd_16() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(Direct(YMM30)), operand2: Some(IndirectDisplaced(RDX, 408359552, Some(OperandSize::Ymmword), None)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: Some(MergeMode::Zero), sae: false, mask: Some(MaskReg::K4), broadcast: None }, &[98, 97, 253, 172, 16, 178, 128, 18, 87, 24], OperandSize::Qword)
}
#[test]
fn vmovupd_17() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(Direct(ZMM0)), operand2: Some(Direct(ZMM1)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: Some(MergeMode::Zero), sae: false, mask: Some(MaskReg::K7), broadcast: None }, &[98, 241, 253, 207, 16, 193], OperandSize::Dword)
}
#[test]
fn vmovupd_18() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(Direct(ZMM0)), operand2: Some(IndirectScaledIndexed(ESI, ECX, Eight, Some(OperandSize::Zmmword), None)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: Some(MergeMode::Zero), sae: false, mask: Some(MaskReg::K4), broadcast: None }, &[98, 241, 253, 204, 16, 4, 206], OperandSize::Dword)
}
#[test]
fn vmovupd_19() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(Direct(ZMM1)), operand2: Some(Direct(ZMM4)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: Some(MergeMode::Zero), sae: false, mask: Some(MaskReg::K6), broadcast: None }, &[98, 241, 253, 206, 16, 204], OperandSize::Qword)
}
#[test]
fn vmovupd_20() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(Direct(ZMM11)), operand2: Some(IndirectScaledDisplaced(RBX, Eight, 1416962940, Some(OperandSize::Zmmword), None)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: Some(MergeMode::Zero), sae: false, mask: Some(MaskReg::K1), broadcast: None }, &[98, 113, 253, 201, 16, 28, 221, 124, 35, 117, 84], OperandSize::Qword)
}
#[test]
fn vmovupd_21() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(Direct(XMM1)), operand2: Some(Direct(XMM5)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: None, sae: false, mask: None, broadcast: None }, &[197, 249, 16, 205], OperandSize::Dword)
}
#[test]
fn vmovupd_22() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(IndirectScaledIndexedDisplaced(EBX, ESI, Four, 270910934, Some(OperandSize::Xmmword), None)), operand2: Some(Direct(XMM0)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: None, sae: false, mask: None, broadcast: None }, &[197, 249, 17, 132, 179, 214, 197, 37, 16], OperandSize::Dword)
}
#[test]
fn vmovupd_23() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(Direct(XMM7)), operand2: Some(Direct(XMM4)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: None, sae: false, mask: None, broadcast: None }, &[197, 249, 16, 252], OperandSize::Qword)
}
#[test]
fn vmovupd_24() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(IndirectScaledIndexedDisplaced(RDI, RSI, Four, 1129257858, Some(OperandSize::Xmmword), None)), operand2: Some(Direct(XMM4)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: None, sae: false, mask: None, broadcast: None }, &[197, 249, 17, 164, 183, 130, 27, 79, 67], OperandSize::Qword)
}
#[test]
fn vmovupd_25() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(Direct(YMM3)), operand2: Some(Direct(YMM7)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: None, sae: false, mask: None, broadcast: None }, &[197, 253, 16, 223], OperandSize::Dword)
}
#[test]
fn vmovupd_26() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(Indirect(EDI, Some(OperandSize::Ymmword), None)), operand2: Some(Direct(YMM6)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: None, sae: false, mask: None, broadcast: None }, &[197, 253, 17, 55], OperandSize::Dword)
}
#[test]
fn vmovupd_27() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(Direct(YMM5)), operand2: Some(Direct(YMM0)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: None, sae: false, mask: None, broadcast: None }, &[197, 253, 16, 232], OperandSize::Qword)
}
#[test]
fn vmovupd_28() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(IndirectDisplaced(RSI, 1989076454, Some(OperandSize::Ymmword), None)), operand2: Some(Direct(YMM5)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: None, sae: false, mask: None, broadcast: None }, &[197, 253, 17, 174, 230, 229, 142, 118], OperandSize::Qword)
}
#[test]
fn vmovupd_29() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(Direct(XMM6)), operand2: Some(Direct(XMM4)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: Some(MergeMode::Zero), sae: false, mask: Some(MaskReg::K2), broadcast: None }, &[98, 241, 253, 138, 16, 244], OperandSize::Dword)
}
#[test]
fn vmovupd_30() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(IndirectScaledIndexedDisplaced(EBX, ESI, Eight, 1711242735, Some(OperandSize::Xmmword), None)), operand2: Some(Direct(XMM2)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: None, sae: false, mask: None, broadcast: None }, &[197, 249, 17, 148, 243, 239, 125, 255, 101], OperandSize::Dword)
}
#[test]
fn vmovupd_31() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(Direct(XMM16)), operand2: Some(Direct(XMM2)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: Some(MergeMode::Zero), sae: false, mask: Some(MaskReg::K3), broadcast: None }, &[98, 225, 253, 139, 16, 194], OperandSize::Qword)
}
#[test]
fn vmovupd_32() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(IndirectDisplaced(RBX, 2001347007, Some(OperandSize::Xmmword), None)), operand2: Some(Direct(XMM24)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: None, sae: false, mask: None, broadcast: None }, &[98, 97, 253, 8, 17, 131, 191, 33, 74, 119], OperandSize::Qword)
}
#[test]
fn vmovupd_33() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(Direct(YMM2)), operand2: Some(Direct(YMM2)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: Some(MergeMode::Zero), sae: false, mask: Some(MaskReg::K5), broadcast: None }, &[98, 241, 253, 173, 16, 210], OperandSize::Dword)
}
#[test]
fn vmovupd_34() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(Indirect(EDX, Some(OperandSize::Ymmword), None)), operand2: Some(Direct(YMM1)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: None, sae: false, mask: None, broadcast: None }, &[197, 253, 17, 10], OperandSize::Dword)
}
#[test]
fn vmovupd_35() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(Direct(YMM1)), operand2: Some(Direct(YMM6)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: Some(MergeMode::Zero), sae: false, mask: Some(MaskReg::K3), broadcast: None }, &[98, 241, 253, 171, 16, 206], OperandSize::Qword)
}
#[test]
fn vmovupd_36() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(IndirectDisplaced(RDI, 23682063, Some(OperandSize::Ymmword), None)), operand2: Some(Direct(YMM30)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: None, sae: false, mask: None, broadcast: None }, &[98, 97, 253, 40, 17, 183, 15, 92, 105, 1], OperandSize::Qword)
}
#[test]
fn vmovupd_37() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(Direct(ZMM0)), operand2: Some(Direct(ZMM7)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: Some(MergeMode::Zero), sae: false, mask: Some(MaskReg::K5), broadcast: None }, &[98, 241, 253, 205, 16, 199], OperandSize::Dword)
}
#[test]
fn vmovupd_38() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(IndirectScaledDisplaced(EAX, Eight, 2015011794, Some(OperandSize::Zmmword), None)), operand2: Some(Direct(ZMM3)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: None, sae: false, mask: None, broadcast: None }, &[98, 241, 253, 72, 17, 28, 197, 210, 163, 26, 120], OperandSize::Dword)
}
#[test]
fn vmovupd_39() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(Direct(ZMM3)), operand2: Some(Direct(ZMM21)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: Some(MergeMode::Zero), sae: false, mask: Some(MaskReg::K5), broadcast: None }, &[98, 177, 253, 205, 16, 221], OperandSize::Qword)
}
#[test]
fn vmovupd_40() {
run_test(&Instruction { mnemonic: Mnemonic::VMOVUPD, operand1: Some(IndirectDisplaced(RDX, 1033804406, Some(OperandSize::Zmmword), None)), operand2: Some(Direct(ZMM10)), operand3: None, operand4: None, lock: false, rounding_mode: None, merge_mode: None, sae: false, mask: None, broadcast: None }, &[98, 113, 253, 72, 17, 146, 118, 154, 158, 61], OperandSize::Qword)
}
| {'content_hash': '7623f27e6c7f5b0023deeedfa91e31ac', 'timestamp': '', 'source': 'github', 'line_count': 208, 'max_line_length': 416, 'avg_line_length': 71.5, 'alnum_prop': 0.6976196880043034, 'repo_name': 'GregoryComer/rust-x86asm', 'id': '3d8ab93fa9770b9b96827f31fd2ecdf71a7467a7', 'size': '14872', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/test/instruction_tests/instr_vmovupd.rs', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Rust', 'bytes': '18282823'}, {'name': 'Shell', 'bytes': '3038'}]} |
using UnityEngine;
using System.Collections;
public class Arrow : MonoBehaviour {
Animator _anim;
Vector3 facing;
public float rayDist;
bool fall;
Vector3 yPos;
public float fallTime;
Transform _myTransform;
Vector3 boxScale;
BoxCollider boxColl;
[HideInInspector]public float damage;
PlayerRanger pr;
GameObject ranger;
void Awake()
{
//_anim.GetComponent<Animator>();
boxColl = GetComponent<BoxCollider>();
boxScale = new Vector3(boxColl.size.x, boxColl.size.y, boxColl.size.z+.2f);
_myTransform = transform;
yPos = new Vector3(0, 1, 0);
transform.up = yPos;
ranger = GameObject.FindWithTag("Ranger");
}
void OnCollisionEnter(Collision other)
{
rigidbody.velocity = Vector3.zero;
fall = true;
}
void OnTriggerEnter (Collider other)
{
if(other.tag == "Ranger")
{
other.GetComponent<PlayerRanger>().arrowsCurr++;
Destroy(gameObject);
}
}
void Update()
{
Debug.DrawRay (transform.position, transform.right * rayDist, Color.magenta);
RaycastHit hit;
if(Physics.Raycast(transform.position, transform.right, out hit, rayDist))
{
if(hit.collider.tag == "Prop")
{
rayDist = 0.015f;
Collection();
}
if(hit.collider.tag == "Warrior")
{
rayDist = 0.015f;
if(!hit.collider.GetComponent<PlayerWarrior>().lockedOn)
{
hit.collider.BroadcastMessage("ApplyDamage", damage);
hit.collider.BroadcastMessage("StopHealthCharge");
ranger.GetComponent<PlayerRanger>().arrowsCurr++;
Destroy(gameObject);
}
}
}
if(fall)
{
rigidbody.useGravity = true;
if(_myTransform.position.y <= -5.94f)
{
rigidbody.velocity = Vector3.zero;
rigidbody.useGravity = false;
Collection();
GetComponent<BoxCollider>().size = boxScale;
fall = false;
}
}
}
void Collection()
{
rigidbody.isKinematic = true;
GetComponent<BoxCollider>().isTrigger = true;
gameObject.layer = LayerMask.NameToLayer("Default");
}
}
| {'content_hash': 'dc3707eb0f56ef03a83c4821ec55f78b', 'timestamp': '', 'source': 'github', 'line_count': 89, 'max_line_length': 79, 'avg_line_length': 21.876404494382022, 'alnum_prop': 0.6825885978428351, 'repo_name': 'Daeltaja/FinalProj', 'id': '8aa416f1cd2b8a73df2b5a78c8e5b3442ce06ebd', 'size': '1949', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'Assets/Scripts/Arrow.cs', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'C', 'bytes': '1725'}, {'name': 'C#', 'bytes': '306121'}, {'name': 'JavaScript', 'bytes': '216'}]} |
/**
* CommonError.java
*
* This file was auto-generated from WSDL
* by the Apache Axis 1.4 Apr 22, 2006 (06:55:48 PDT) WSDL2Java emitter.
*/
package com.google.api.ads.dfp.v201302;
/**
* A place for common errors that can be used across services.
*/
public class CommonError extends com.google.api.ads.dfp.v201302.ApiError implements java.io.Serializable {
private com.google.api.ads.dfp.v201302.CommonErrorReason reason;
public CommonError() {
}
public CommonError(
java.lang.String fieldPath,
java.lang.String trigger,
java.lang.String errorString,
java.lang.String apiErrorType,
com.google.api.ads.dfp.v201302.CommonErrorReason reason) {
super(
fieldPath,
trigger,
errorString,
apiErrorType);
this.reason = reason;
}
/**
* Gets the reason value for this CommonError.
*
* @return reason
*/
public com.google.api.ads.dfp.v201302.CommonErrorReason getReason() {
return reason;
}
/**
* Sets the reason value for this CommonError.
*
* @param reason
*/
public void setReason(com.google.api.ads.dfp.v201302.CommonErrorReason reason) {
this.reason = reason;
}
private java.lang.Object __equalsCalc = null;
public synchronized boolean equals(java.lang.Object obj) {
if (!(obj instanceof CommonError)) return false;
CommonError other = (CommonError) obj;
if (obj == null) return false;
if (this == obj) return true;
if (__equalsCalc != null) {
return (__equalsCalc == obj);
}
__equalsCalc = obj;
boolean _equals;
_equals = super.equals(obj) &&
((this.reason==null && other.getReason()==null) ||
(this.reason!=null &&
this.reason.equals(other.getReason())));
__equalsCalc = null;
return _equals;
}
private boolean __hashCodeCalc = false;
public synchronized int hashCode() {
if (__hashCodeCalc) {
return 0;
}
__hashCodeCalc = true;
int _hashCode = super.hashCode();
if (getReason() != null) {
_hashCode += getReason().hashCode();
}
__hashCodeCalc = false;
return _hashCode;
}
// Type metadata
private static org.apache.axis.description.TypeDesc typeDesc =
new org.apache.axis.description.TypeDesc(CommonError.class, true);
static {
typeDesc.setXmlType(new javax.xml.namespace.QName("https://www.google.com/apis/ads/publisher/v201302", "CommonError"));
org.apache.axis.description.ElementDesc elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("reason");
elemField.setXmlName(new javax.xml.namespace.QName("https://www.google.com/apis/ads/publisher/v201302", "reason"));
elemField.setXmlType(new javax.xml.namespace.QName("https://www.google.com/apis/ads/publisher/v201302", "CommonError.Reason"));
elemField.setMinOccurs(0);
elemField.setNillable(false);
typeDesc.addFieldDesc(elemField);
}
/**
* Return type metadata object
*/
public static org.apache.axis.description.TypeDesc getTypeDesc() {
return typeDesc;
}
/**
* Get Custom Serializer
*/
public static org.apache.axis.encoding.Serializer getSerializer(
java.lang.String mechType,
java.lang.Class _javaType,
javax.xml.namespace.QName _xmlType) {
return
new org.apache.axis.encoding.ser.BeanSerializer(
_javaType, _xmlType, typeDesc);
}
/**
* Get Custom Deserializer
*/
public static org.apache.axis.encoding.Deserializer getDeserializer(
java.lang.String mechType,
java.lang.Class _javaType,
javax.xml.namespace.QName _xmlType) {
return
new org.apache.axis.encoding.ser.BeanDeserializer(
_javaType, _xmlType, typeDesc);
}
}
| {'content_hash': '1f209feaa3c66ee2a4a2c7d6b18158de', 'timestamp': '', 'source': 'github', 'line_count': 133, 'max_line_length': 135, 'avg_line_length': 30.834586466165412, 'alnum_prop': 0.6100950987564009, 'repo_name': 'google-code-export/google-api-dfp-java', 'id': '0c2a22760e7b7c87e715a51d332992ee25d88fcf', 'size': '4101', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/com/google/api/ads/dfp/v201302/CommonError.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Java', 'bytes': '39950935'}]} |
package net.sargue.mailgun;
import javax.ws.rs.client.*;
import java.util.List;
/**
* Representation of a Mailgun's mail request.
* <p>
* It must be built using a {@link MailBuilder}.
*/
public abstract class Mail {
private final Configuration configuration;
Mail(Configuration configuration) {
this.configuration = configuration;
}
/**
* Convenience shortcut to {@code MailBuilder.using(configuration)}
*
* @param configuration the configuration to use
* @return a new {@link MailBuilder} which uses the specified configuration
*/
public static MailBuilder using(Configuration configuration) {
return new MailBuilder(configuration);
}
/**
* Retrieves the value of a given mail parameter. If there are multiple
* values the first one is returned. If the parameter hasn't been set
* null is returned.
*
* Can only be used on simple parameters (String). So don't use it on
* <i>attachment</i> for example. Doing so will throw a
* {@link IllegalStateException}.
*
* @param param the name of the parameter
* @return the first value of the parameter, if any, null otherwise
* @throws IllegalStateException if the parameter is not a simple (basic text) one
*/
public abstract String getFirstValue(String param);
/**
* Retrieves the values of a given mail parameter. If the parameter hasn't
* been set an empty list is returned.
*
* Can only be used on simple parameters (String). So don't use it on
* <i>attachment</i> for example. Doing so will throw a
* {@link IllegalStateException}.
*
* @param param the name of the parameter
* @return the list of values for the parameter or an empty list
* @throws IllegalStateException if the parameter is not a simple (basic text) one
*/
public abstract List<String> getValues(String param);
/**
* Sends the email.
* <p>
* This method send the request to the Mailgun service. It is a
* <strong>blocking</strong> method so it will return upon request
* completion.
*
* @return the response from the Mailgun service or null if the message
* is not sent (filtered by {@link MailSendFilter}
*/
public Response send() {
if (!configuration.mailSendFilter().filter(this)) return null;
prepareSend();
return new Response(request().post(entity()));
}
/**
* Sends the email asynchronously.
* <p>
* This method returns immediately, sending the request to the Mailgun
* service in the background. It is a <strong>non-blocking</strong>
* method.
*
* @param callback the callback to be invoked upon completion or failure
*/
public void sendAsync(final MailRequestCallback callback) {
if (!configuration.mailSendFilter().filter(this)) return;
prepareSend();
request()
.async()
.post(entity(),
new InvocationCallback<javax.ws.rs.core.Response>() {
@Override
public void completed(javax.ws.rs.core.Response o) {
callback.completed(new Response(o));
}
@Override
public void failed(Throwable throwable) {
callback.failed(throwable);
}
});
}
/**
* Sends the email asynchronously. It uses the configuration provided
* default callback if available, ignoring the outcome otherwise.
*
* If you want to use a specific callback for this call use
* {@link #sendAsync(MailRequestCallback)} instead.
*/
public void sendAsync() {
if (!configuration.mailSendFilter().filter(this)) return;
MailRequestCallbackFactory factory = configuration.mailRequestCallbackFactory();
if (factory == null) {
prepareSend();
request().async().post(entity());
} else
sendAsync(factory.create(this));
}
/**
* Retrieves the configuration associated with this Mail.
*
* @return the underlying configuration
*/
public Configuration configuration() {
return configuration;
}
abstract Entity<?> entity(); //NOSONAR
abstract void prepareSend();
void configureTarget(WebTarget target) {
//defaults to no-op
}
private Invocation.Builder request() {
WebTarget target = configuration.getTarget();
configureTarget(target);
return target.path(configuration.domain()).path("messages").request();
}
}
| {'content_hash': '37c2b2a7556228c212197762bba63646', 'timestamp': '', 'source': 'github', 'line_count': 140, 'max_line_length': 88, 'avg_line_length': 33.93571428571428, 'alnum_prop': 0.6162913070932435, 'repo_name': 'sargue/mailgun', 'id': 'a40874e02801c190fb9de545fc637f6d2c250388', 'size': '4751', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'src/main/java/net/sargue/mailgun/Mail.java', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'Java', 'bytes': '117011'}]} |
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical" >
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="50dp"
android:background="#484E61"
>
<TextView
android:id="@+id/title_text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:textColor="#fff"
android:textSize="24sp"
/>
</RelativeLayout>
<ListView
android:id="@+id/list_view"
android:layout_width="match_parent"
android:layout_height="match_parent"
>
</ListView>
</LinearLayout>
| {'content_hash': '8f579652f42173c3e02ab7db9ae0706d', 'timestamp': '', 'source': 'github', 'line_count': 29, 'max_line_length': 72, 'avg_line_length': 30.75862068965517, 'alnum_prop': 0.5964125560538116, 'repo_name': 'yb1415185602/collweather', 'id': '658826ca8d5a0494bfc6b4e5b8c7fdf3b969b075', 'size': '892', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'res/layout/choose_area.xml', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Java', 'bytes': '27177'}]} |
<?php
use yii\helpers\Html;
use yii\widgets\ActiveForm;
use yii\helpers\ArrayHelper;
use kartik\select2\Select2;
/* @var $this yii\web\View */
/* @var $model app\models\Empleado */
/* @var $form yii\widgets\ActiveForm */
?>
<div class="empleado-form">
<?php $form = ActiveForm::begin(); ?>
<?= $form->field($model, 'nombre')->textInput() ?>
<?= $form->field($model, 'direccion')->textInput() ?>
<?= $form->field($model, 'contacto')->textInput() ?>
<?= $form->field($model, 'telefono')->textInput() ?>
<?= $form->field($model, 'correo')->textInput() ?>
<?= Html::submitButton($model->isNewRecord ? 'Guardar' : 'Guardar cambios', ['class' => $model->isNewRecord ? 'btn btn-success' : 'btn btn-primary']) ?>
</div>
<?php ActiveForm::end(); ?>
</div>
| {'content_hash': 'eae12c72c212f0a39e8bc9b915f06a96', 'timestamp': '', 'source': 'github', 'line_count': 34, 'max_line_length': 160, 'avg_line_length': 23.558823529411764, 'alnum_prop': 0.5930087390761548, 'repo_name': 'rzamarripa/shabel', 'id': '2baa8926b064eb2a287b05a5e1cdd226cbe8c53f', 'size': '801', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'views/proveedor/_form.php', 'mode': '33188', 'license': 'bsd-3-clause', 'language': [{'name': 'ApacheConf', 'bytes': '199'}, {'name': 'Batchfile', 'bytes': '1030'}, {'name': 'CSS', 'bytes': '1533204'}, {'name': 'HTML', 'bytes': '179629'}, {'name': 'JavaScript', 'bytes': '3469599'}, {'name': 'PHP', 'bytes': '295872'}]} |
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.gr4yscale.havit.MainActivity">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!" />
</RelativeLayout>
| {'content_hash': 'ef328dcd460b0dc0dd7a198ee8c2140c', 'timestamp': '', 'source': 'github', 'line_count': 16, 'max_line_length': 74, 'avg_line_length': 43.6875, 'alnum_prop': 0.7267525035765379, 'repo_name': 'gr4yscale/havit', 'id': 'ee547d115bf31a18531fca906258dac19bc0fae1', 'size': '699', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'android/Havit/app/src/main/res/layout/activity_main.xml', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'C', 'bytes': '6911'}, {'name': 'Java', 'bytes': '6698'}, {'name': 'JavaScript', 'bytes': '131667'}, {'name': 'Objective-C', 'bytes': '236235'}, {'name': 'Shell', 'bytes': '50'}]} |
// -*- mode: C++; c-indent-level: 4; c-basic-offset: 4; indent-tabs-mode: nil; -*-
//
// rcast.h: Rcpp R/C++ interface class library -- cast from one SEXP type to another
//
// Copyright (C) 2010 - 2013 Dirk Eddelbuettel and Romain Francois
//
// This file is part of Rcpp.
//
// Rcpp is free software: you can redistribute it and/or modify it
// under the terms of the GNU General Public License as published by
// the Free Software Foundation, either version 2 of the License, or
// (at your option) any later version.
//
// Rcpp is distributed in the hope that it will be useful, but
// WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
// You should have received a copy of the GNU General Public License
// along with Rcpp. If not, see <http://www.gnu.org/licenses/>.
#ifndef Rcpp_rcast_h
#define Rcpp_rcast_h
#include <Rcpp/exceptions.h>
namespace Rcpp{
namespace internal {
inline SEXP convert_using_rfunction(SEXP x, const char* const fun){
Armor<SEXP> res ;
try{
SEXP funSym = Rf_install(fun);
res = Rcpp_eval( Rf_lang2( funSym, x ) ) ;
} catch( eval_error& e){
throw not_compatible( std::string("could not convert using R function : ") + fun ) ;
}
return res;
}
// r_true_cast is only meant to be used when the target SEXP type
// is different from the SEXP type of x
template <int TARGET>
SEXP r_true_cast( SEXP x) {
throw not_compatible( "not compatible" ) ;
return x ; // makes solaris happy
}
template <int RTYPE>
SEXP basic_cast( SEXP x){
if( TYPEOF(x) == RTYPE ) return x ;
switch( TYPEOF(x) ){
case REALSXP:
case RAWSXP:
case LGLSXP:
case CPLXSXP:
case INTSXP:
return Rf_coerceVector( x, RTYPE) ;
default:
throw ::Rcpp::not_compatible( "not compatible with requested type" ) ;
}
return R_NilValue ; /* -Wall */
}
template<>
inline SEXP r_true_cast<INTSXP>(SEXP x){
return basic_cast<INTSXP>(x) ;
}
template<>
inline SEXP r_true_cast<REALSXP>(SEXP x){
return basic_cast<REALSXP>(x) ;
}
template<>
inline SEXP r_true_cast<RAWSXP>(SEXP x){
return basic_cast<RAWSXP>(x) ;
}
template<>
inline SEXP r_true_cast<CPLXSXP>(SEXP x){
return basic_cast<CPLXSXP>(x) ;
}
template<>
inline SEXP r_true_cast<LGLSXP>(SEXP x){
return basic_cast<LGLSXP>(x) ;
}
template <>
inline SEXP r_true_cast<STRSXP>(SEXP x){
switch( TYPEOF( x ) ){
case CPLXSXP:
case RAWSXP:
case LGLSXP:
case REALSXP:
case INTSXP:
{
// return Rf_coerceVector( x, STRSXP );
// coerceVector does not work for some reason
Shield<SEXP> call( Rf_lang2( Rf_install( "as.character" ), x ) ) ;
Shield<SEXP> res( Rcpp_eval( call, R_GlobalEnv ) ) ;
return res ;
}
case CHARSXP:
return Rf_ScalarString( x ) ;
case SYMSXP:
return Rf_ScalarString( PRINTNAME( x ) ) ;
default:
throw ::Rcpp::not_compatible( "not compatible with STRSXP" ) ;
}
return R_NilValue ; /* -Wall */
}
template<>
inline SEXP r_true_cast<VECSXP>(SEXP x) {
return convert_using_rfunction(x, "as.list" ) ;
}
template<>
inline SEXP r_true_cast<EXPRSXP>(SEXP x) {
return convert_using_rfunction(x, "as.expression" ) ;
}
template<>
inline SEXP r_true_cast<LISTSXP>(SEXP x) {
switch( TYPEOF(x) ){
case LANGSXP:
{
Shield<SEXP> y( Rf_duplicate( x ));
SET_TYPEOF(y,LISTSXP) ;
return y ;
}
default:
return convert_using_rfunction(x, "as.pairlist" ) ;
}
}
template<>
inline SEXP r_true_cast<LANGSXP>(SEXP x) {
return convert_using_rfunction(x, "as.call" ) ;
}
} // namespace internal
template <int TARGET> SEXP r_cast(SEXP x) {
if (TYPEOF(x) == TARGET) {
return x;
} else {
#ifdef RCPP_WARN_ON_COERCE
Shield<SEXP> result( internal::r_true_cast<TARGET>(x) );
Rf_warning("coerced object from '%s' to '%s'",
CHAR(Rf_type2str(TYPEOF(x))),
CHAR(Rf_type2str(TARGET))
);
return result;
#else
return internal::r_true_cast<TARGET>(x);
#endif
}
}
} // namespace Rcpp
#endif
| {'content_hash': 'dc9b692f6bde56c1053394b14f140621', 'timestamp': '', 'source': 'github', 'line_count': 157, 'max_line_length': 101, 'avg_line_length': 32.93630573248408, 'alnum_prop': 0.5167279056275382, 'repo_name': 'bccpp/bccpp.github.io', 'id': '496a4d9965cca71e2bcd40a5c9ef2bd8f6fd096c', 'size': '5171', 'binary': False, 'copies': '2', 'ref': 'refs/heads/master', 'path': 'packrat/lib/x86_64-w64-mingw32/3.3.2/Rcpp/include/Rcpp/r_cast.h', 'mode': '33188', 'license': 'apache-2.0', 'language': []} |
package org.apache.geronimo.connector;
import java.util.Timer;
import javax.resource.spi.UnavailableException;
import javax.resource.spi.XATerminator;
import javax.resource.spi.work.WorkManager;
import org.apache.geronimo.connector.work.GeronimoWorkManager;
/**
* GBean BootstrapContext implementation that refers to externally configured WorkManager
* and XATerminator gbeans.
*
* @version $Rev$ $Date$
*/
public class BootstrapContextImpl implements javax.resource.spi.BootstrapContext {
private final GeronimoWorkManager workManager;
/**
* Normal constructor for use as a GBean.
* @param workManager
*/
public BootstrapContextImpl(final GeronimoWorkManager workManager) {
this.workManager = workManager;
}
/**
* @see javax.resource.spi.BootstrapContext#getWorkManager()
*/
public WorkManager getWorkManager() {
return workManager;
}
/**
* @see javax.resource.spi.BootstrapContext#getXATerminator()
*/
public XATerminator getXATerminator() {
return workManager.getXATerminator();
}
/**
* @see javax.resource.spi.BootstrapContext#createTimer()
*/
public Timer createTimer() throws UnavailableException {
return new Timer();
}
// public static final GBeanInfo GBEAN_INFO;
//
// static {
// GBeanInfoBuilder infoFactory = new GBeanInfoBuilder(BootstrapContext.class);
// //adding interface does not work, creates attributes for references???
//// infoFactory.addInterface(javax.resource.spi.BootstrapContext.class);
//
// infoFactory.addOperation("createTimer");
// infoFactory.addOperation("getWorkManager");
// infoFactory.addOperation("getXATerminator");
//
// infoFactory.addReference("WorkManager", WorkManager.class);
// infoFactory.addReference("XATerminator", XATerminator.class);
//
// infoFactory.setConstructor(new String[]{"WorkManager", "XATerminator"});
//
// GBEAN_INFO = infoFactory.getBeanInfo();
// }
//
// public static GBeanInfo getGBeanInfo() {
// return GBEAN_INFO;
// }
}
| {'content_hash': 'fd0a1148f0563b637e757c4619efa2bf', 'timestamp': '', 'source': 'github', 'line_count': 75, 'max_line_length': 89, 'avg_line_length': 28.466666666666665, 'alnum_prop': 0.6899297423887588, 'repo_name': 'vibe13/geronimo', 'id': '28ddbdfa9ff250192460eb02a3f700b9f772ee6a', 'size': '2765', 'binary': False, 'copies': '2', 'ref': 'refs/heads/1.0', 'path': 'modules/connector/src/java/org/apache/geronimo/connector/BootstrapContextImpl.java', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'Batchfile', 'bytes': '29627'}, {'name': 'CSS', 'bytes': '47972'}, {'name': 'HTML', 'bytes': '838469'}, {'name': 'Java', 'bytes': '8975734'}, {'name': 'JavaScript', 'bytes': '906'}, {'name': 'Shell', 'bytes': '32814'}, {'name': 'XSLT', 'bytes': '4468'}]} |
/*
Name: Base16 Atelier Dune Light
Author: Bram de Haan (http://atelierbram.github.io/syntax-highlighting/atelier-schemes/dune)
Pygments template by Jan T. Sott (https://github.com/idleberg)
Created with Base16 Builder by Chris Kempson (https://github.com/chriskempson/base16-builder)
*/
.highlight .hll { background-color: #e8e4cf }
.highlight { color: #6E6B5E; }
.highlight .c { color: #999580 } /* Comment */
.highlight .err { color: #d73737 } /* Error */
.highlight .k { color: #b854d4 } /* Keyword */
.highlight .l { color: #b65611 } /* Literal */
.highlight .n { color: #6E6B5E; } /* Name */
.highlight .o { color: #1fad83 } /* Operator */
.highlight .p { color: #6E6B5E; } /* Punctuation */
.highlight .cm { color: #999580 } /* Comment.Multiline */
.highlight .cp { color: #999580 } /* Comment.Preproc */
.highlight .c1 { color: #999580 } /* Comment.Single */
.highlight .cs { color: #999580 } /* Comment.Special */
.highlight .gd { color: #d73737 } /* Generic.Deleted */
.highlight .ge { font-style: italic } /* Generic.Emph */
.highlight .gh { color: #20201d; font-weight: bold } /* Generic.Heading */
.highlight .gi { color: #60ac39 } /* Generic.Inserted */
.highlight .gp { color: #999580; font-weight: bold } /* Generic.Prompt */
.highlight .gs { font-weight: bold } /* Generic.Strong */
.highlight .gu { color: #1fad83; font-weight: bold } /* Generic.Subheading */
.highlight .kc { color: #b854d4 } /* Keyword.Constant */
.highlight .kd { color: #b854d4 } /* Keyword.Declaration */
.highlight .kn { color: #1fad83 } /* Keyword.Namespace */
.highlight .kp { color: #b854d4 } /* Keyword.Pseudo */
.highlight .kr { color: #b854d4 } /* Keyword.Reserved */
.highlight .kt { color: #ae9513 } /* Keyword.Type */
.highlight .ld { color: #60ac39 } /* Literal.Date */
.highlight .m { color: #b65611 } /* Literal.Number */
.highlight .s { color: #2A9292; } /* Literal.String */
.highlight .na { color: #6684e1 } /* Name.Attribute */
.highlight .nb { color: #B65611; } /* Name.Builtin */
.highlight .nc { color: #ae9513 } /* Name.Class */
.highlight .no { color: #d73737 } /* Name.Constant */
.highlight .nd { color: #1fad83 } /* Name.Decorator */
.highlight .ni { color: #20201d } /* Name.Entity */
.highlight .ne { color: #d73737 } /* Name.Exception */
.highlight .nf { color: #6684e1 } /* Name.Function */
.highlight .nl { color: #20201d } /* Name.Label */
.highlight .nn { color: #ae9513 } /* Name.Namespace */
.highlight .nx { color: #6684e1 } /* Name.Other */
.highlight .py { color: #20201d } /* Name.Property */
.highlight .nt { color: #1fad83 } /* Name.Tag */
.highlight .nv { color: #d73737 } /* Name.Variable */
.highlight .ow { color: #1fad83 } /* Operator.Word */
.highlight .w { color: #20201d } /* Text.Whitespace */
.highlight .mf { color: #b65611 } /* Literal.Number.Float */
.highlight .mh { color: #b65611 } /* Literal.Number.Hex */
.highlight .mi { color: #b65611 } /* Literal.Number.Integer */
.highlight .mo { color: #b65611 } /* Literal.Number.Oct */
.highlight .sb { color: #60ac39 } /* Literal.String.Backtick */
.highlight .sc { color: #20201d } /* Literal.String.Char */
.highlight .sd { color: #999580 } /* Literal.String.Doc */
.highlight .s2 { color: #60ac39 } /* Literal.String.Double */
.highlight .se { color: #b65611 } /* Literal.String.Escape */
.highlight .sh { color: #60ac39 } /* Literal.String.Heredoc */
.highlight .si { color: #b65611 } /* Literal.String.Interpol */
.highlight .sx { color: #60ac39 } /* Literal.String.Other */
.highlight .sr { color: #60ac39 } /* Literal.String.Regex */
.highlight .s1 { color: #60ac39 } /* Literal.String.Single */
.highlight .ss { color: #60ac39 } /* Literal.String.Symbol */
.highlight .bp { color: #CD0101 } /* Name.Builtin.Pseudo */
.highlight .vc { color: #d73737 } /* Name.Variable.Class */
.highlight .vg { color: #d73737 } /* Name.Variable.Global */
.highlight .vi { color: #d73737 } /* Name.Variable.Instance */
.highlight .il { color: #b65611 } /* Literal.Number.Integer.Long */
| {'content_hash': '896ebb0d0fed348fdd4cc99a7dd7489b', 'timestamp': '', 'source': 'github', 'line_count': 71, 'max_line_length': 100, 'avg_line_length': 56.25352112676056, 'alnum_prop': 0.6479719579369053, 'repo_name': 'starsep/rust-www', 'id': 'c10c63c767699b7345d1a22b35890dfa72ee1ecf', 'size': '3994', 'binary': False, 'copies': '6', 'ref': 'refs/heads/master', 'path': 'css/syntax-highlight.css', 'mode': '33188', 'license': 'apache-2.0', 'language': [{'name': 'CSS', 'bytes': '46168'}, {'name': 'HTML', 'bytes': '217249'}, {'name': 'JavaScript', 'bytes': '15235'}, {'name': 'Python', 'bytes': '1195'}, {'name': 'Ruby', 'bytes': '806'}, {'name': 'Rust', 'bytes': '5235'}, {'name': 'Shell', 'bytes': '78'}]} |
export default {
newConference: '新しい会議',
dialInNumber: 'ダイヤルイン番号',
host: 'ホスト',
participants: '参加者',
internationalParticipants: '海外の参加者',
internationalNumbersHeader: '国際ダイヤルイン番号の選択',
search: '検索...',
inviteWithText: 'テキストで招待',
inviteText: '{brandName}会議に参加してください\u3002\r\n\r\nダイヤルイン番号\uFF1A{formattedDialInNumber} \r\n{additionalNumbersSection} \r\n参加者のアクセス\uFF1A{participantCode} \r\n\r\n国際ダイヤルイン番号が必要な場合は\u3001次をご覧ください\uFF1A{dialInNumbersLinks} \r\n\r\nこの電話会議は\u3001{brandName} Conferencingを使用して開催されています\u3002',
hostAccess: 'ホストのアクセス',
participantsAccess: '参加者のアクセス',
addinalDialInNumbers: '追加のダイヤルイン番号',
selectNumbers: '番号の選択',
enableJoinBeforeHost: 'ホストより前の参加を可能にする',
conferenceCommands: '会議コマンド',
inviteWithGCalendar: 'Googleカレンダーを使用して招待',
joinAsHost: '会議を起動',
internationalNumber: '国際ダイヤルイン番号\uFF1A',
};
// @key: @#@"newConference"@#@ @source: @#@"New Conference"@#@
// @key: @#@"dialInNumber"@#@ @source: @#@"Dial-in Number"@#@
// @key: @#@"hostAccess"@#@ @source: @#@"Host Access"@#@
// @key: @#@"participantsAccess"@#@ @source: @#@"Participants Access"@#@
// @key: @#@"addinalDialInNumbers"@#@ @source: @#@"Additional Dial-in Numbers"@#@
// @key: @#@"selectNumbers"@#@ @source: @#@"Select Numbers"@#@
// @key: @#@"enableJoinBeforeHost"@#@ @source: @#@"Enable join before Host"@#@
// @key: @#@"conferenceCommands"@#@ @source: @#@"Conference Commands"@#@
// @key: @#@"inviteWithGCalendar"@#@ @source: @#@"Invite with Google Calendar"@#@
// @key: @#@"inviteWithText"@#@ @source: @#@"Invite with Text"@#@
// @key: @#@"joinAsHost"@#@ @source: @#@"Launch Conference"@#@
// @key: @#@"internationalNumber"@#@ @source: @#@"International Dial-in Numbers:"@#@
// @key: @#@"inviteText_att"@#@ @source: @#@"Please join the {brandName} conference.\n\nDial-In Number: {formattedDialInNumber} \n{additionalNumbersSection} \nParticipant Access: {participantCode} \n\nNeed an international dial-in phone number? Please visit {dialInNumbersLinks} \n\nThis conference call is brought to you by {brandName} Conferencing."@#@
// @key: @#@"inviteText_bt"@#@ @source: @#@"Please join the {brandName} conference.\n\nDial-In Number: {formattedDialInNumber} \n{additionalNumbersSection} \nParticipant Access: {participantCode} \n\nAdditional dial-in numbers {dialInNumbersLinks}"@#@
// @key: @#@"inviteText_rc"@#@ @source: @#@"Please join the {brandName} conference.\n\nDial-In Number: {formattedDialInNumber} \n{additionalNumbersSection} \nParticipant Access: {participantCode} \n\nNeed an international dial-in phone number? Please visit {dialInNumbersLinks} \n\nThis conference call is brought to you by {brandName} Conferencing."@#@
// @key: @#@"inviteText_telus"@#@ @source: @#@"Please join the {brandName} conference.\n\nDial-In Number: {formattedDialInNumber} \n{additionalNumbersSection} \nParticipant Access: {participantCode} \n\nAdditional dial-in numbers {dialInNumbersLinks}"@#@
| {'content_hash': 'fb9e78995c5b94bc89f88d12455d46de', 'timestamp': '', 'source': 'github', 'line_count': 37, 'max_line_length': 354, 'avg_line_length': 78.67567567567568, 'alnum_prop': 0.7124699416008244, 'repo_name': 'u9520107/ringcentral-js-widget', 'id': '9a1069fb22239d4014bc1bd79928c1b619fd2b80', 'size': '3309', 'binary': False, 'copies': '1', 'ref': 'refs/heads/master', 'path': 'packages/ringcentral-widgets/components/ConferencePanel/i18n/ja-JP.js', 'mode': '33188', 'license': 'mit', 'language': [{'name': 'CSS', 'bytes': '90533'}, {'name': 'HTML', 'bytes': '2967'}, {'name': 'JavaScript', 'bytes': '433434'}, {'name': 'Shell', 'bytes': '1001'}]} |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.