path
stringlengths 7
265
| concatenated_notebook
stringlengths 46
17M
|
---|---|
EDA and data visualization of Land use with Seaborn.ipynb | ###Markdown
Exploratory Data Analysis and visualisation of UN FAO Land use data with Seaborn 1) Python package and data import
###Code
%matplotlib inline
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
import seaborn as sns
pd.set_option('display.max_rows', 500)
pd.set_option('display.max_columns', None)
landuse = pd.read_csv('landuse_clean.csv')
landuse.head(4)
landuse.shape
landuse.info()
landuse['Country'].nunique() # number of unique country names in the DataFrame 'Country' column
landuse['Country'].unique() # list of unique country names
###Output
_____no_output_____
###Markdown
2) Sub-selection of landuse DataFrame Create 6 lists: 1) list that leaves only the countries 2) List of countries grouped by continental region 3) World region 4) Economic type 5) Continent 6) World: total data for all countries.
###Code
not_countries_list = ['Africa', 'Asia', 'Americas', 'Australia and New Zealand','Belgium-Luxembourg',
'Caribbean','Central America','Northern America','South America',
'Eastern Africa','Middle Africa','Northern Africa','Southern Africa','Western Africa',
'Central Asia','Eastern Asia','Western Asia','Southern Asia','South-Eastern Asia',
'Eastern Europe', 'Europe','European Union','Northern Europe','Southern Europe',
'Western Europe','Polynesia','Melanesia','Micronesia','Oceania','Least Developed Countries',
'Land Locked Developing Countries','Small Island Developing States',
'Low Income Food Deficit Countries',
'Net Food Importing Developing Countries','World']
Cont_region_list = ['Caribbean','Central America','Northern America','South America',
'Eastern Africa','Middle Africa','Northern Africa','Southern Africa','Western Africa',
'Central Asia','Eastern Asia','Western Asia','Southern Asia','South-Eastern Asia',
'Eastern Europe', 'Northern Europe','Southern Europe',
'Western Europe','Polynesia','Melanesia','Micronesia','Oceania']
World_region_list =['Americas', 'Africa', 'Asia', 'Europe','Oceania']
Econ_area_list = ['European Union','Least Developed Countries','Land Locked Developing Countries','Small Island Developing States',
'Low Income Food Deficit Countries','Net Food Importing Developing Countries']
Continent_list = ['Africa', 'Northern America','South America', 'Asia', 'Europe', 'Australia']
World = ['World']
###Output
_____no_output_____
###Markdown
2.1) Create Countries DataFrame
###Code
countries = landuse.loc[~landuse['Country'].isin(not_countries_list)]
#Use ~ to negate the boolean,selects rows that are not in the list.
countries.head(100)
countries.shape
#countries['Country'].unique() # unique returns unique values as a numpy array
###Output
_____no_output_____
###Markdown
Save countries data frame for making a choropleth
###Code
countries.to_csv('countries_land_use.csv', index=False);
###Output
_____no_output_____
###Markdown
2.2) Create Continent Regions DataFrame
###Code
cont_regions = landuse.loc[landuse['Country'].isin(Cont_region_list)]
cont_regions['Country'].unique()
cont_regions.shape
###Output
_____no_output_____
###Markdown
2.3) Continents DataFrames
###Code
continents = landuse.loc[landuse['Country'].isin(Continent_list)]
continents['Country'].unique()
continents.shape
###Output
_____no_output_____
###Markdown
2.4) Create econ Dataframe
###Code
econ = landuse.loc[landuse['Country'].isin(Econ_area_list)]
econ.shape
econ['Country'].unique()
econ.head(3)
###Output
_____no_output_____
###Markdown
2.5) Create world region dataframe
###Code
World_region_list =['Americas', 'Africa', 'Asia', 'Europe','Oceania']
world_reg = landuse.loc[landuse['Country'].isin(World_region_list)]
world_reg['Country'].unique()
###Output
_____no_output_____
###Markdown
2.6) Create World DataFrameFrom the Country column select values that are in the World list, which is just World.
###Code
world = landuse.loc[landuse['Country'].isin(World)]
world.head(3)
world.shape
###Output
_____no_output_____
###Markdown
3) Melt and reshape each of the DataFrames
###Code
landuse.head(3)
landuse_melt = pd.melt(frame = landuse, id_vars =['Country', 'Land_use', 'Element', 'Unit'],var_name='year', value_name='hectares')
landuse_sorted =landuse_melt.sort_values(by=['Land_use', 'year'])
landuse_sorted.head(8)
landuse_melt.head(2)
###Output
_____no_output_____
###Markdown
Use pivot_table to create a DataFrame with land use variables as columns and each row is a individual obeservation for land use in a specific country for a specific year
###Code
landuse_cols = landuse_melt.pivot_table(index=['Country','year'], columns='Land_use', values='hectares').reset_index()
landuse_cols.head(2)
world.shape
world_melt = pd.melt(frame = world, id_vars =['Country', 'Land_use', 'Element', 'Unit'],
var_name='year', value_name='hectares')
world_melt.head(2)
world_pivot = world_melt.pivot_table(index=['Country','year'], columns='Land_use', values='hectares')
world_cols = world_pivot.reset_index()
world_cols
world_cols.columns
world_reg_melt = pd.melt(frame = world_reg, id_vars =['Country', 'Land_use', 'Element', 'Unit'],
var_name='year', value_name='hectares')
world_reg_melt
world_reg_pivot = world_reg_melt.pivot_table(index=['Country','year'], columns='Land_use', values='hectares')
world_reg_cols = world_reg_pivot.reset_index()
world_reg_cols
countries_melt = pd.melt(frame = countries, id_vars =['Country', 'Land_use', 'Element', 'Unit'],
var_name='year', value_name='hectares')
countries_melt
countries_pivot = countries_melt.pivot_table(index=['Country','year'], columns='Land_use', values='hectares')
countries_cols = countries_pivot.reset_index()
countries_cols
cont_regions_melt = pd.melt(frame = cont_regions, id_vars =['Country', 'Land_use', 'Element', 'Unit'],
var_name='year', value_name='hectares')
cont_regions_pivot = cont_regions_melt.pivot_table(index=['Country','year'], columns='Land_use', values='hectares')
cont_regions_cols = cont_regions_pivot.reset_index()
cont_regions_cols
continents_melt = pd.melt(frame = continents, id_vars =['Country', 'Land_use', 'Element', 'Unit'],
var_name='year', value_name='hectares')
continents_melt
continents_pivot = continents_melt.pivot_table(index=['Country','year'], columns='Land_use', values='hectares')
continents_cols = continents_pivot.reset_index()
continents_cols
econ_melt = pd.melt(frame = econ, id_vars =['Country', 'Land_use', 'Element', 'Unit'],
var_name='year', value_name='hectares')
econ_pivot = econ_melt.pivot_table(index=['Country','year'], columns='Land_use', values='hectares')
econ_cols = econ_pivot.reset_index()
econ_cols
###Output
_____no_output_____
###Markdown
4) Data visualisation DataFrames world, world_melt, world_cols world_reg, world_reg_melt, world_reg_cols countries, countries_melt, countries_cols cont_regions, cont_regions_melt, cont_regions_cols continents, continents_melt, continents_cols econ, econ_melt, econ_cols What variables do we want to look at to examine food production globally? Cropland combines "Arable land" and "Permanent crops". "Arable land" combines land under temporary crops (<1 year growing cycle, newly sown after the harvest), temporary meadows and pastures (cultivated with herbaceous forage crops for mowing and pasture for period of <5 year), and land with temporary fallow. Land under Permanent crops is land cultivated with long-term crops which do not have to be replanted for several years (e.g. cocoa and coffee), land under trees and shrubs producing flowers, and nurseries (except those for forest trees, which are classed under "Forestry"). Land under Permanent meadows and pastures is land used permanently (five years or more) to grow herbaceous forage crops through cultivation or naturally (wild prairie or grazing land). To get a detailed picture of food production we will use the more granular variables rather than "Cropland". Examining the Non-Null Count below for Arable and the individual temporary variables shows that Arable has 14991 non-null values versus 3192 and 5643 non-null values for the temporary variables. So we will use Arable land for temporary land use measures. Land under permanent medows and crops are >14,000. Not all land use measures are recorded for every area. But Arable Land, Land under perm. meadows and pastures and Land under permanent crops are recorded for all sub-dataframes.
###Code
#landuse_cols.info()
###Output
_____no_output_____
###Markdown
4.1) Analysis of world level data
###Code
world_cols.columns
###Output
_____no_output_____
###Markdown
Create a subset of the DataFrame with columns of interest
###Code
world_cols_cp = world_cols[['Country','Land area','year','Arable land',
'Land under perm. meadows and pastures','Land under permanent crops']]
type(world_cols_cp)
###Output
_____no_output_____
###Markdown
Distribution plotCreate a Distplot to look at the distribution of hectares for each land use type from 1961-2017. Setting kde=False creates a histogram for each of the land use variables.
###Code
sns.set_style('ticks')
sns.set_context('notebook')
fig, (ax0, ax1, ax2) = plt.subplots(nrows=3, ncols=1, sharey=True, figsize=(7,10))
sns.distplot(world_cols_cp['Arable land'], ax=ax0, kde=False,rug=True, axlabel='Arable land (ha)', bins=10, color='brown')
ax0.set_ylabel('Frequency')
sns.distplot(world_cols_cp['Land under perm. meadows and pastures'],ax=ax1, kde=False, rug=True,
axlabel='Permanent meadows & pastures (ha)', bins=10, color='green')
ax1.set_ylabel('Frequency')
sns.distplot(world_cols_cp['Land under permanent crops'],ax=ax2, hist=True, kde=False, rug=True,axlabel='Permanent crops (ha)',
bins=10, color='orange')
ax2.set_ylabel('Frequency')
sns.despine() #remove top and left box boundary
plt.tight_layout(pad=1.0)
plt.show()
###Output
_____no_output_____
###Markdown
Relational plots and Categorical plots
###Code
melt_world_cols_cp = pd.melt(frame=world_cols_cp, id_vars =['Country', 'Land area', 'year'],
var_name='Land use', value_name='hectares')
melt_world_cols_cp.head(2)
###Output
_____no_output_____
###Markdown
Create a new column for land use as a percentage of global land area
###Code
melt_world_cols_cp['hect_pct'] = 100 * melt_world_cols_cp['hectares']/melt_world_cols_cp['Land area']
melt_world_cols_cp.head(2)
cp_palette = ['brown', 'green', 'orange']
g=sns.catplot(x='Land use', y='hectares', data = melt_world_cols_cp, aspect=1.4, kind='bar', palette=cp_palette)
g.set(ylabel='Global Land use', xlabel='')
g.set_xticklabels(['Arable', 'Perm meadows & pastures', 'Permanent crops'], size =12)
g.set_yticklabels(size=12)
h=sns.catplot(x='Land use', y='hect_pct', data = melt_world_cols_cp, aspect=1.4, kind='bar',palette=cp_palette)
h.set(ylabel='Land use as a % of global land area', xlabel='')
h.set_xticklabels(['Arable', 'Perm meadows & pastures', 'Permanent crops'], size=12)
h.set_yticklabels(size=12)
plt.subplots_adjust(hspace= 0.3)
plt.show()
###Output
_____no_output_____
###Markdown
Boxplot
###Code
g=sns.catplot(x='Land use', y='hectares', data=melt_world_cols_cp, kind='box', aspect=1.5, palette=cp_palette)
g.fig.suptitle('World land use from 1961 to 2017', y=1.03)
g.set(ylabel='Hectares', xlabel='')
g.set_xticklabels(['Arable', 'Perm meadows & pastures', 'Permanent crops'])
plt.show()
###Output
_____no_output_____
###Markdown
relplot lineplot
###Code
sns.set(style="ticks")
g=sns.relplot(x='year', y='hectares', data=melt_world_cols_cp, kind='line',hue='Land use', aspect=1.5, palette=cp_palette)
g.set(ylabel='Hectares', xlabel='')
g.fig.suptitle("Global land use", y=1.03)
#A typical way of changing the location of a legend in matplotlib is to use the arguments loc and bbox_to_anchor.
#In Seaborn's relplot a FacetGrid object is returned. In order to get the legend object we can use ._legend.
#We can then set the bbox_to_anchor
g._legend.set_bbox_to_anchor([1,0.6])
g.set(xticks=melt_world_cols_cp.year[0::3]) # start at 0 and show every third tick label
plt.xticks(rotation=60)
plt.tight_layout(pad=2.0)
plt.show()
###Output
_____no_output_____
###Markdown
4.2) Continent level analysis
###Code
cont_cols_cp = continents_cols[['Country','Land area','year','Arable land',
'Land under perm. meadows and pastures','Land under permanent crops']]
melt_cont_cols_cp = pd.melt(cont_cols_cp, id_vars=['Country', 'Land area', 'year'],
var_name='Land use', value_name='hectares')
melt_cont_cols_cp
melt_cont_cols_cp['hect_pct'] = 100 * melt_cont_cols_cp['hectares']/melt_cont_cols_cp['Land area']
melt_cont_cols_cp.head(20)
#continent=sns.color_palette('hls',8)
#sns.palplot(continent)
cust_cont = ['crimson', 'darkorange','greenyellow','forestgreen',
'cyan','blue']
sns.set_palette(cust_cont)
sns.palplot(cust_cont)
###Output
_____no_output_____
###Markdown
Swarmplot
###Code
sns.set_style('whitegrid')
g=sns.stripplot(data=melt_cont_cols_cp, y='Land use', x='hectares', hue='Country',jitter=True, dodge=True, palette=cust_cont)
g.set(xlabel='Hectares', ylabel='')
g.set_yticklabels(['Arable', 'Perm meadows & pastures', 'Permanent crops'])
plt.legend(bbox_to_anchor=(1.05, 1), loc=2, borderaxespad=0.)
plt.show()
# top plot dodge=True, bottom plot dodge=False
sns.set_style('whitegrid')
fig, (ax0, ax1) = plt.subplots(nrows=2, ncols=1, figsize=(6,10))
sns.swarmplot(data=melt_cont_cols_cp, y='Land use', x='hectares',hue='Country', dodge=True, palette=cust_cont, ax=ax0)
ax0.set(xlabel='Land use (ha), 1961-2017', ylabel='')
ax0.set_yticklabels(['Arable', 'Perm meadows & pastures', 'Permanent crops'])
ax0.legend(bbox_to_anchor=(1.05, 1), loc=2, borderaxespad=0.)
sns.swarmplot(data=melt_cont_cols_cp, y='Land use', x='hect_pct',hue='Country', dodge=False, palette=cust_cont, ax=ax1)
ax1.set(xlabel='Land use as % of continent land area, 1961-2017', ylabel='')
ax1.set_yticklabels(['Arable', 'Perm meadows & pastures', 'Permanent crops'])
ax1.legend(bbox_to_anchor=(1.05, 1), loc=2, borderaxespad=0.)
fig.suptitle("Continental land use from 1961-2017", y=0.91)
plt.subplots_adjust(hspace=0.3)
plt.show()
sns.set(style='ticks')
f=sns.relplot(x='year', y='hectares', data=melt_cont_cols_cp, kind='scatter',hue='Country',row='Land use', height=4, aspect=3,
palette=cust_cont)
f.set(ylabel='Hectares', xlabel='')
f.fig.suptitle("Continental land use from 1961-2017", y=1.03)
f.set_xticklabels(rotation=90)
plt.subplots_adjust(hspace=0.25)
plt.show()
###Output
_____no_output_____
###Markdown
4.3) Country level analysis Analyse countries at continent level.Plotting data for 240 countries is unyieldy. Clearly some continents have the largest proportion of arable land and in some continents the amount of land in agricultural use has increased. Interestingly for the majority of continents land use has remained constant, despite world population growing. If we want to carry out a detailed analysis at country level, subdividing the dataframe by continent would be an efficient approach. To do this we will have to add a column for continent to the countries_cols DataFrame. We'll add the column to the entire countries DataFrame not just the sub-dataframe for arable and permanent pastures, as it might be useful to have it for analysis of other variables later on, such as forestry etc.For each country in the countries_cols dataframe we have to assign a continent label in a new column. We can use Pandas map() function to do this. There are a few steps we have to complete before using the map function. 4.3.1) Dictionaries from lists and DataFrame .query() A) Create lists of countries in each continent. These lists will be used to create a dictionary of values for each continent that can be mapped to each country in the dataframe.
###Code
Asia=['Afghanistan','Armenia','Azerbaijan',
'Bahrain', 'Bangladesh','Bhutan', 'Brunei Darussalam',
'Cambodia', 'China', 'China, Hong Kong SAR', 'China, Macao SAR',
'China, Taiwan Province of', 'China, mainland','Cyprus',
"Democratic People's Republic of Korea",
'Georgia', 'India', 'Indonesia', 'Iran (Islamic Republic of)',
'Iraq', 'Israel','Japan', 'Jordan', 'Kazakhstan', 'Kuwait',
'Kyrgyzstan', "Lao People's Democratic Republic",
'Lebanon', 'Malaysia','Maldives', 'Mongolia', 'Myanmar',
'Nepal', 'Oman','Pakistan','Palestine','Philippines',
'Qatar','Republic of Korea', 'Saudi Arabia','Singapore',
'Sri Lanka', 'Syrian Arab Republic', 'Tajikistan',
'Thailand', 'Timor-Leste', 'Turkey', 'Turkmenistan',
'United Arab Emirates', 'Uzbekistan', 'Viet Nam','Yemen']
Africa = ['Algeria','Angola',
'Benin', 'Botswana', 'Burkina Faso', 'Burundi',
'Cabo Verde', 'Cameroon','Central African Republic', 'Chad', 'Comoros', 'Congo',
"Côte d'Ivoire",'Democratic Republic of the Congo', 'Djibouti',
'Egypt', 'Equatorial Guinea', 'Eritrea','Eswatini', 'Ethiopia', 'Ethiopia PDR',
'Gabon', 'Gambia','Ghana', 'Guinea','Guinea-Bissau',
'Kenya', 'Lesotho', 'Liberia', 'Libya', 'Madagascar',
'Malawi','Mali','Mauritania', 'Mauritius', 'Mayotte', 'Morocco', 'Mozambique',
'Namibia','Niger', 'Nigeria','Réunion','Rwanda',
'Saint Helena, Ascension and Tristan da Cunha','Sao Tome and Principe','Senegal', 'Seychelles',
'Sierra Leone', 'Somalia', 'South Africa', 'South Sudan','Sudan', 'Sudan (former)',
'Togo', 'Tunisia', 'Uganda', 'United Republic of Tanzania', 'Western Sahara',
'Zambia', 'Zimbabwe']
Europe = ['Albania','Andorra','Austria',
'Belarus','Belgium', 'Bosnia and Herzegovina','Bulgaria',
'Channel Islands','Croatia','Czechia', 'Czechoslovakia',
'Denmark', 'Estonia','Faroe Islands','Finland',
'France', 'Germany','Gibraltar', 'Greece', 'Hungary',
'Iceland', 'Ireland', 'Isle of Man', 'Italy', 'Latvia',
'Liechtenstein','Lithuania', 'Luxembourg', 'Malta', 'Montenegro',
'Netherlands', 'North Macedonia', 'Norway', 'Poland', 'Portugal',
'Republic of Moldova', 'Romania', 'Russian Federation',
'San Marino', 'Serbia', 'Serbia and Montenegro', 'Slovakia', 'Slovenia',
'Spain', 'Sweden', 'Switzerland', 'Ukraine','United Kingdom','USSR',
'Yugoslav SFR']
Australia = ['Australia', 'New Zealand', 'Norfolk Island']
Northern_America = ['Bermuda','Canada','Greenland','Saint Pierre and Miquelon','United States of America']
South_America = ['Argentina','Bolivia (Plurinational State of)','Brazil','Chile','Colombia',
'Ecuador','Falkland Islands (Malvinas)', 'French Guiana','Guyana','Paraguay','Peru',
'Suriname','Uruguay','Venezuela (Bolivarian Republic of)']
###Output
_____no_output_____
###Markdown
B) Change the lists into dictionaries using dict.fromkeys(seq, 'value')The first argument is seq, the list of values that will be used as the dictionary keys (key = country in country list above). The second argument is value and is the value of the key, value = continent Inputting a single value for the value argument means that all values in the key:value pairs will be the same. Using the dictionary we map the continent value to the countries as a new column.
###Code
#Change each list into a dictionary
Asia_dict = dict.fromkeys(Asia, 'Asia')
Africa_dict = dict.fromkeys(Africa, 'Africa')
Europe_dict = dict.fromkeys(Europe, 'Europe')
Aus_dict = dict.fromkeys(Australia, 'Australia')
North_America_dict = dict.fromkeys(Northern_America, 'Northern America')
South_America_dict = dict.fromkeys(South_America, 'South America')
Asia_dict
###Output
_____no_output_____
###Markdown
Merge the 6 dictionaries into a single County_cont_dict.
###Code
# Merge all the dictionaries into one dictionary
Country_cont_dict = {**Asia_dict, **Africa_dict, **Europe_dict, ** Aus_dict, **North_America_dict,**South_America_dict}
countries_cols['Continent'] = countries_cols['Country'].map(Country_cont_dict)
###Output
_____no_output_____
###Markdown
Create a new column 'Continent' in the countries_cols DataFrame and use the map() function to map the key from the dictionary to the corresponding 'Country' value in the countries_cols DataFrame. The key value is then added to the continent column.
###Code
countries_cols.head()
coun_cols_cp = countries_cols[['Continent','Country','Land area','year','Arable land',
'Land under perm. meadows and pastures','Land under permanent crops']]
melt_coun_cols_cp = pd.melt(coun_cols_cp, id_vars=['Continent','Country', 'Land area', 'year'],
var_name='Land use', value_name='hectares')
melt_coun_cols_cp.head(2)
#number of individual countries in the DataFrame.
#Not all countries have a continent label as smaller islands are not included in the continent lists,
#such as islands in thh Oceania region.
num=coun_cols_cp['Country']
num.nunique(dropna=False)
coun_cols_cp.describe()
###Output
_____no_output_____
###Markdown
Scatterplot
###Code
fig, ax = plt.subplots(figsize=(20,15))
sns.set_context('talk')
sns.scatterplot(x='Land area', y='hectares',data=melt_coun_cols_cp,hue='Country',palette= 'tab20', style='Land use',ax=ax,s=100)
ax.set(xlabel='Land area (ha)', ylabel='Hectares')
ax.legend(loc='upper left', bbox_to_anchor=(0, -0.1),borderaxespad=0.,ncol=8, fontsize=14)
plt.show()
###Output
_____no_output_____
###Markdown
relplot scatterplot
###Code
sns.set_context('notebook')
g=sns.relplot(x='year', y='hectares', data=melt_coun_cols_cp, kind='scatter', row='Land use',
size='Land area', hue='Land area', aspect=2.0, palette='RdYlGn')
g.set(xlabel='')
g.set_xticklabels(rotation=90)
plt.subplots_adjust(hspace=0.3)
melt_coun_cols_cp.head(2)
###Output
_____no_output_____
###Markdown
Customised color palettes
###Code
Asia_country=['dimgrey', 'lightgrey', 'rosybrown','lightcoral','firebrick','red','mistyrose','coral','orangered', 'chocolate','saddlebrown',
'peachpuff','peru','darkorange','tan','goldenrod','gold','khaki', 'darkkhaki','olive','yellow','olivedrab','yellowgreen',
'greenyellow','darkseagreen','lightgreen','forestgreen','lime','aquamarine','mediumturquoise','lightcyan','teal','aqua','powderblue',
'deepskyblue','lightskyblue','steelblue','dodgerblue','cornflowerblue','royalblue','lavender','midnightblue','blue','darkslateblue','mediumslateblue',
'mediumpurple','rebeccapurple','indigo','darkorchid','thistle','hotpink','purple']
#'violet','magenta','orchid','mediumvioletred','hotpink','lavenderblush','palevioletred','pink']
Africa_country=['dimgrey', 'lightgrey', 'rosybrown','lightcoral','firebrick','red','mistyrose','coral','orangered', 'chocolate','saddlebrown',
'peachpuff','peru','darkorange','tan','goldenrod','gold','khaki', 'darkkhaki','olive','yellow','olivedrab','yellowgreen',
'greenyellow','darkseagreen','lightgreen','forestgreen','lime','aquamarine','mediumturquoise','lightcyan','teal','aqua','powderblue',
'deepskyblue','lightskyblue','steelblue','dodgerblue','cornflowerblue','royalblue','lavender','midnightblue','blue','darkslateblue','mediumslateblue',
'mediumpurple','rebeccapurple','indigo','darkorchid','thistle','hotpink','purple','violet','magenta','orchid',
'mediumvioletred','hotpink','lavenderblush','palevioletred','pink']
#sns.palplot(cust_country)
#60 colours in palette
#60 countries in Africa DF
#52 countries in Asia DF
country=sns.color_palette('plasma',52)
sns.palplot(country)
count = sns.husl_palette(52, h=.5)
sns.palplot(count)
###Output
_____no_output_____
###Markdown
Select countries from each continent using .query() to plot on scatter plots grouped by land use
###Code
plot = sns.relplot(x='Land area', y='hectares', data=melt_coun_cols_cp.query('Continent =="Asia"'),
kind='scatter',col='Land use',col_wrap = 2, hue = 'Country',
size='Land area',sizes=(50,200),legend='brief', aspect=1, palette=Asia_country)
plot.fig.suptitle('Land use in Asia from 1961 - 2017', x=0.5, y=1.025)
#g._legend.set_title('title')
# remove the default legend and create a separate legend object that can be located using bbox_to_anchor
handles,labels = plot.axes[0].get_legend_handles_labels()
plot._legend.remove()
plot.fig.legend(handles, labels, ncol=3, bbox_to_anchor=(1.20, 0.47), frameon=False);
#placing ; at the end of the last line of code removes the line of text printed above the plot
pal1=['orangered','peru','gold','khaki','darkkhaki','navy','deepskyblue','dimgrey',
'lightskyblue','lime','indigo','thistle']
plot = sns.relplot(x='year', y='hectares', data=melt_coun_cols_cp.query('Continent=="Asia" & hectares > 25000'),
kind='line',col='Land use', col_wrap=1,hue = 'Country',legend='brief', aspect=2, palette=pal1)
plt.xticks(rotation=90)
plt.subplots_adjust(hspace=0.3);
plot = sns.relplot(x='Land area', y='hectares', data=melt_coun_cols_cp.query('Continent =="Africa"'),
kind='scatter',col='Land use',col_wrap = 2, hue = 'Country',
size='Land area',sizes=(50,200),
legend='brief', aspect=1, palette=Africa_country)
plot.fig.suptitle('Land use in Africa from 1961 - 2017', x=0.5, y=1.025)
handles,labels = plot.axes[0].get_legend_handles_labels()
plot._legend.remove()
plot.fig.legend(handles, labels, ncol=3, bbox_to_anchor=(1.015, 0.49),
frameon=False);
pal2=['orangered','peru','gold','khaki','darkkhaki','navy','deepskyblue','dimgrey',
'lightskyblue','lime','indigo','thistle']
plot2 = sns.relplot(x='year', y='hectares', data=melt_coun_cols_cp.query('Continent=="Africa" & hectares>50000'),
kind='line',col='Land use', col_wrap=1,hue = 'Country',legend='brief', aspect=2)
plt.xticks(rotation=90);
#plt.subplots_adjust(hspace=0.3);
plot = sns.relplot(x='Land area', y='hectares', data=melt_coun_cols_cp.query('Continent =="Europe"'),
kind='scatter',col='Land use',col_wrap = 2, hue = 'Country',
size='Land area',sizes=(50,200),alpha=0.5,
legend='brief', aspect=1)
plot.fig.suptitle('Land use in Europe from 1961 - 2017', x=0.5, y=1.025)
handles,labels = plot.axes[0].get_legend_handles_labels()
plot._legend.remove()
plot.fig.legend(handles, labels, ncol=3, bbox_to_anchor=(0.98, 0.47),
frameon=False);
pal1=['orangered','peru','gold','khaki','darkkhaki','navy','deepskyblue','dimgrey',
'lightskyblue','lime','indigo','thistle']
plot = sns.relplot(x='year', y='hectares', data=melt_coun_cols_cp.query('Continent=="Europe" & hectares > 50000'),
kind='line',col='Land use', col_wrap=1,hue = 'Country',legend='brief', aspect=2)
plt.xticks(rotation=90)
plt.subplots_adjust(hspace=0.3);
USSR = melt_coun_cols_cp[melt_coun_cols_cp['Country']=="USSR"]
USSR
plot = sns.relplot(x='Land area', y='hectares', data=melt_coun_cols_cp.query('Continent =="South America"'),
kind='scatter',col='Land use',col_wrap = 2, hue = 'Country',
size='Land area',sizes=(50,200),
legend='brief', aspect=1)
plot.fig.suptitle('Land use in South America from 1961 - 2017', x=0.5, y=1.025)
handles,labels = plot.axes[0].get_legend_handles_labels()
plot._legend.remove()
plot.fig.legend(handles, labels, ncol=1, bbox_to_anchor=(0.7, 0.5),
frameon=False);
pal1=['orangered','peru','gold','khaki','darkkhaki','navy','deepskyblue','dimgrey',
'lightskyblue','lime','indigo','thistle']
plot = sns.relplot(x='year', y='hectares', data=melt_coun_cols_cp.query('Continent=="South America" & hectares > 20000'),
kind='line',col='Land use', col_wrap=1,hue = 'Country',legend='brief', aspect=2)
plt.xticks(rotation=90)
plt.subplots_adjust(hspace=0.3);
plot = sns.relplot(x='Land area', y='hectares', data=melt_coun_cols_cp.query('Continent =="Northern America"'),
kind='scatter',col='Land use',col_wrap = 2, hue = 'Country',
size='Land area',sizes=(50,200),
legend='brief', aspect=1)
plot.fig.suptitle('Land use in North America from 1961 - 2017', x=0.5, y=1.025)
handles,labels = plot.axes[0].get_legend_handles_labels()
plot._legend.remove()
plot.fig.legend(handles, labels, ncol=1, bbox_to_anchor=(0.7, 0.4),
frameon=False);
plot = sns.relplot(x='Land area', y='hectares', data=melt_coun_cols_cp.query('Continent =="Australia"'),
kind='scatter',col='Land use',col_wrap = 2, hue = 'Country',
size='Land area',sizes=(50,200),
legend='brief', aspect=1)
plot.fig.suptitle('Land use in Australia from 1961 - 2017', x=0.5, y=1.025)
handles,labels = plot.axes[0].get_legend_handles_labels()
plot._legend.remove()
plot.fig.legend(handles, labels, ncol=1, bbox_to_anchor=(0.7, 0.4),
frameon=False);
###Output
_____no_output_____
###Markdown
Plot countries in which the amount fo land area in use has changed over time
###Code
pal3 = ['orangered','peru','gold','darkkhaki','darkgreen','navy','deepskyblue','dimgrey',
'lightskyblue','lime','indigo']
sns.set_style('whitegrid')
plot = sns.relplot(x='Land area', y='hectares',
data=melt_coun_cols_cp.query('Country ==["India", "China","Saudi Arabia","Mongolia", "Sudan(former)","South Africa", "USSR", "Russian Federation", "Brazil", "Argentina","United States of America", "Australia"]'),
kind='scatter',col='Land use',col_wrap = 2, hue = 'Country', size='Land area',sizes=(50,200),
legend='brief', aspect=1, palette=pal3)
plot.fig.suptitle('Countries in which land use area has changed since 1961', x=0.46, y=1.028, fontsize=14)
handles,labels = plot.axes[0].get_legend_handles_labels()
plot._legend.remove()
plot.fig.legend(handles, labels, ncol=1, bbox_to_anchor=(0.7, 0.46),frameon=False);
pal3 = ['orangered','peru','gold','darkkhaki','darkgreen','navy','deepskyblue','dimgrey','lightskyblue','lime','indigo']
sns.set_style('whitegrid')
plot = sns.relplot(x='year', y='hectares',
data=melt_coun_cols_cp.query('Country ==["India", "China", "Saudi Arabia","Mongolia","Sudan(former)","South Africa", "USSR", "Russian Federation", "Brazil", "Argentina","United States of America", "Australia"]'),
kind='scatter',col='Land use', col_wrap=1,hue = 'Country',legend='brief', aspect=2, palette=pal3)
plt.xticks(rotation=90)
plt.subplots_adjust(hspace=0.3)
plot.fig.suptitle('Countries in which land use area has changed since 1961', x=0.46, y=1.028, fontsize=14);
###Output
_____no_output_____
###Markdown
Average land use hectares
###Code
Mean_hect = melt_coun_cols_cp.groupby(['Land use','Country']).mean()
Mean_hect.sort_values(['hectares'], ascending=False).head(100)
sns.set_style('whitegrid')
country_pal=['lightgreen','orangered','peru','gold','darkkhaki','darkgreen','lime', 'red','navy','deepskyblue','dimgrey','lightskyblue','indigo']
plot = sns.catplot(x='hectares', y='Country',
data=melt_coun_cols_cp.query('Country ==["Australia", "China", "United States of America", "Brazil", "India", "Mongolia", "Saudi Arabia", "Argentina","Sudan(former)","Kazakhstan","South Africa","Mexico","Russian Federation","Angola"]'),
kind='point',col='Land use', join=False, ci='sd', hue='Country', legend=False, palette=country_pal)
plot.set(ylabel='', xlabel='Hectares');
###Output
_____no_output_____ |
notebooks/data_cleanup.ipynb | ###Markdown
1. Load
###Code
import pandas as pd
import numpy as np
import geopy
import us
df = pd.read_csv("../data/Mass Shootings Dataset Ver 2.csv", encoding = "ISO-8859-1")
df.replace([np.NaN], [''], inplace=True)
df.columns
###Output
_____no_output_____
###Markdown
2. Process Title
###Code
df.Title.head()
###Output
_____no_output_____
###Markdown
Location
###Code
df.Location.replace(['Washington D.C.', ''],
['Washington, Washington', ','],
inplace=True)
cities, raw_states = zip(*[value.rsplit(',', maxsplit=1) for value in df.Location.values])
df['Cities'] = cities
states = [us.states.lookup(raw_state.strip()).name if raw_state else '' for raw_state in raw_states]
df['State'] = states
###Output
_____no_output_____
###Markdown
examples of multiple cities per one location:
###Code
for location in df.Location.unique():
if len(location.split(',')) != 2:
print(location)
df.drop('Location', axis=1, inplace=True)
###Output
_____no_output_____
###Markdown
Date
###Code
df.Date.head()
###Output
_____no_output_____
###Markdown
Summary
###Code
df.Summary.head()
###Output
_____no_output_____
###Markdown
Fatalities
###Code
df.Fatalities.unique()
###Output
_____no_output_____
###Markdown
Injured
###Code
df.Injured.unique()
###Output
_____no_output_____
###Markdown
Total victims
###Code
df['Total victims'].unique()
all(df.Fatalities.values + df.Injured.values == df['Total victims'].values)
###Output
_____no_output_____
###Markdown
Total victims inconsistency:
###Code
for f, i, t, _ in zip(df.Fatalities.values, df.Injured.values, df['Total victims'].values, range(50)):
if f + i != t:
print(f, '+', i, '!=', t)
###Output
1 + 4 != 4
0 + 3 != 4
3 + 1 != 3
2 + 2 != 3
2 + 2 != 3
2 + 2 != 3
4 + 0 != 3
4 + 0 != 3
2 + 2 != 3
5 + 0 != 4
4 + 14 != 17
###Markdown
possible differences:
###Code
np.unique(df.Fatalities.values + df.Injured.values - df['Total victims'].values)
###Output
_____no_output_____
###Markdown
Mental Health Issues
###Code
df['Mental Health Issues'].unique()
df['Mental Health Issues'].replace(['Unclear', 'Unknown', 'unknown'],
['Unknown', 'Unknown', 'Unknown'], inplace=True)
df['Mental Health Issues'].unique()
###Output
_____no_output_____
###Markdown
Race
###Code
df.Race.unique()
df.Race.replace(['', 'Other', 'Black American or African American',
'White American or European American', 'Asian American',
'Some other race', 'Two or more races',
'Black American or African American/Unknown',
'White American or European American/Some other Race',
'Native American or Alaska Native', 'white', 'black',
'Asian American/Some other race'],
['Unknown', 'Unknown', 'Black', 'White', 'Asian',
'Unknown', 'Mixed', 'Black', 'White', 'Native',
'White', 'Black', 'Asian'],
inplace=True)
df.Race.unique()
###Output
_____no_output_____
###Markdown
Gender
###Code
df.Gender.unique()
df.Gender.replace(['Male', 'Male/Female', 'Female', 'M/F'],
['M', 'Unknown', 'F', 'Unknown'],
inplace=True)
df.Gender.unique()
###Output
_____no_output_____
###Markdown
Latitude
###Code
df.Latitude.head()
###Output
_____no_output_____
###Markdown
Longitude
###Code
df.Longitude.head()
###Output
_____no_output_____
###Markdown
Fill missing states based on coordinates
###Code
missing_state_latitudes = df.Latitude[df.State == ''].values
missing_state_longitudes = df.Longitude[df.State == ''].values
geotagger = geopy.GoogleV3()
def coordinates_to_state_name(latitude, longitude):
try:
query = f'{latitude}, {longitude}'
result = geotagger.reverse(query)
address_elements = result[1].address.split(',')
state_string = address_elements[-2]
state_code = state_string.split()[0]
state_name = us.states.lookup(state_code).name
return state_name
except IndexError:
print('Missing state name', result)
except Exception:
print('Bad request', query)
return ''
missing_state_names = [
coordinates_to_state_name(latitude, longitude)
for latitude, longitude in zip(missing_state_latitudes, missing_state_longitudes)
]
df.State[df.State == ''] = missing_state_names
###Output
/home/maciej/miniconda3/envs/ed/lib/python3.6/site-packages/ipykernel_launcher.py:1: SettingWithCopyWarning:
A value is trying to be set on a copy of a slice from a DataFrame
See the caveats in the documentation: http://pandas.pydata.org/pandas-docs/stable/indexing.html#indexing-view-versus-copy
"""Entry point for launching an IPython kernel.
###Markdown
Gun laws http://lawcenter.giffords.org/scorecard/A - the strongest gun lawsF - the weakest gun laws
###Code
gun_laws = pd.read_csv("../data/gun_laws.csv", encoding="UTF-8")
gun_laws.columns
df = df.merge(gun_laws, on='State')
###Output
_____no_output_____
###Markdown
Party affiliation http://www.pewforum.org/religious-landscape-study/compare/party-affiliation/by/state/
###Code
party_affiliation = pd.read_csv("../data/party_affiliation.csv", encoding="UTF-8")
party_affiliation.columns
df_pa = df.merge(party_affiliation, on='State')
republican = df_pa['Republican lean'] > df_pa['Democrat lean']
democrat = df_pa['Democrat lean'] > df_pa['Republican lean']
df['Party affiliation'] = 'Neutral'
df['Party affiliation'][democrat] = 'Democrat'
df['Party affiliation'][republican] = 'Republican'
set(party_affiliation.State) - set(df.State)
###Output
_____no_output_____
###Markdown
Population
###Code
population = pd.read_csv("../data/population.csv", encoding="UTF-8")
population.columns
df = df.merge(population, on='State')
###Output
_____no_output_____
###Markdown
3. Save
###Code
df.drop('Summary', axis=1, inplace=True)
df.to_csv("../data/Mass Shootings Dataset Ver 2 clean.csv", encoding = "ISO-8859-1", index=False)
###Output
_____no_output_____ |
Gaussian-processes/GPR.ipynb | ###Markdown
A TUTORIAL ON GAUSSIAN PROCESS REGRESSIONby Sebastian T. Glavind, May, 2020, May, 2021
###Code
import numpy as np
import math
import GPy
import scipy.stats as ss
import scipy.special as ssp
import seaborn as sns
import pandas as pd
import pickle
from matplotlib import pyplot as plt
%matplotlib inline
###Output
_____no_output_____
###Markdown
Single-output Gaussian Process RegressionSee Rasmussen and Williams (2006) for a reference on Gaussian processes. The modelIn this section, we consider the following definition for a regression of some response variable $y$ based on covariate(s) $\mathbf{x}$:$$y = f(\mathbf{x}) + \epsilon,$$where we assume that the observed $y$'s differ from the functional values $f(\mathbf{x})$ by additive noise $\epsilon$. In the following sections, we show how the functional relationship $f$ may be established by use of Gaussian process regression. First, we introduce Gaussian processes in a single output setting (this section); and second, we proceed to cover the multi-output setting (next section).We start by assuming that $f$ is a non-linear, non-parametric function with a GP prior:$$f(\mathbf{x}) \sim \mathcal{GP}(m(\mathbf{x}), k(\mathbf{x}, \mathbf{x}')),$$where $m(\mathbf{x}) = \mathbb{E}[f(\mathbf{x})]$ is the expected value function, and $k(\mathbf{x},\mathbf{x}') = \text{cov}[f(\mathbf{x}), f(\mathbf{x}')]$ is the positive definite covariance or kernel function.Given a data set $\mathcal{D} = \{ \mathbf{X}, \mathbf{y} \} = \{ \mathbf{x}[n], y[n] \}_{n=1}^N$ of (potentially) vector-valued inputs and scalar outputs, we construct the GP prior by evaluating the mean and covariance function at the data points, which leads to a multivariate Gaussian distribution over the corresponding function values:$$f(\mathbf{X}) \sim \mathcal{N}(m(\mathbf{X}),k(\mathbf{X},\mathbf{X})), $$where $f(\mathbf{X})=\{ f(\mathbf{x}[n]) \}_{n=1}^N$. Under proper normalization of the data, the expected value of the process can be assumed to be zero without lose of generality, and the covariance function should then capture basic aspects of the process, such as stationarity and smoothness.If we further assume the noise process to be Gaussian white noise, the output observations $\mathbf{y}$ and the functional evaluation $f(\mathbf{x}_{\star})$ at a new test point $\mathbf{x}_{\star}$ are jointly Gaussian, and by application of the standard rules for conditioning of Gaussian distributed random variables, we arrive at a closed-form expression for the predictive distribution of $f(\mathbf{x}_{\star})$:$$ p(f(\mathbf{x}_{\star})|\mathcal{D},\mathbf{x}_{\star}, \Theta) = \mathcal{N}(f_{\star}(\mathbf{x}_{\star}), k_{\star}(\mathbf{x}_{\star}, \mathbf{x}_{\star}) ), $$where $\Theta$ denotes the set of model parameters, and $f_{\star}$ and $k_{\star}$ are defined as$$ f_{\star}(\mathbf{x}_{\star}) = \mathbf{k}_{\mathbf{x}_{\star}}^T (k(\mathbf{X}, \mathbf{X}) + \sigma^2\mathbf{I} )^{-1} \mathbf{y}, $$$$ k_{\star}(\mathbf{x}_{\star}, \mathbf{x}_{\star}) = k(\mathbf{x}_{\star},\mathbf{x}_{\star}) - \mathbf{k}_{\mathbf{x}_{\star}}^T (k(\mathbf{X}, \mathbf{X}) + \sigma^2\mathbf{I} )^{-1} \mathbf{k}_{\mathbf{x}_{\star}} $$with $\mathbf{k}_{\mathbf{x}_{\star}}$ as a shorthand notation for $k(\mathbf{X},\mathbf{x}_{\star})$, and $\mathbf{I}$ is the identity matrix.***C. E. Rasmussen, C. K. Williams, Gaussian processes for machine learning, MIT press, 2006.*** Sample dataThe example in this tutorial is inspired by Katherine Bailey's excellent, introductory blog post on GP regression: https://katbailey.github.io/post/gaussian-processes-for-dummies/.
###Code
# Noise free/Noisy training data
np.random.seed(42)
nX_tr = 20 # no. of training points
std_y = 1e-1 # if noise free set to 1e-4 for numerical stability; corr. var = 1e-16 addition along diagonal
Xtrain = np.random.uniform(low=-4, high=1, size=(nX_tr,1)).reshape(-1,1) # input
ytrain = np.sin(Xtrain) + std_y*np.random.normal(size=(nX_tr,1)) # output (sin function)
# Test data
nX_te = 50 # no. of test points
Xtest = np.linspace(-5, 5, nX_te).reshape(-1,1) # input
ytest = np.sin(Xtest) + std_y*np.random.normal(size=(nX_te,1)) # output (sin function)
# plot
plt.plot(Xtrain, ytrain, 'bs', ms=6);
plt.plot(Xtest, ytest, 'gs', ms=3);
plt.xlabel('x');
plt.ylabel('y');
plt.title('Training and test set');
###Output
_____no_output_____
###Markdown
Kernel functionWe will study the squared exponential kernel, which for one-dimensional input reads$$ k(x,x') = \sigma_f^2 \exp\left( - \frac{(x-x')^2}{2 l^2} \right) $$
###Code
# Kernel function: Squared exponential (SE)
# Param is length scale squared
def se_kern(x, y, sig_f, l_f):
sqdist = np.sum(x**2,1).reshape(-1,1) + np.sum(y**2,1) - 2*np.dot(x, y.T) # reshape(-1,1): from one-dim. to two-dim. array.
K = sig_f**2 * np.exp( -0.5 * sqdist / (l_f**2) )
return(K)
# # test implementation - OK
# def se_kern2(x, y, sig_f, l_f):
# n1 = x.shape[0]
# n2 = y.shape[0]
# K = np.zeros((n1,n2))
# for i in range(n1):
# for j in range(n2):
# K[i,j] = sig_f**2 * np.exp( -0.5 * (x[i]-y[j])**2 / (l_f**2) )
# return(K)
# kk1=se_kern(Xtrain, ytrain, 1., 2.)
# kk2=se_kern2(Xtrain, ytrain, 1., 2.)
# (np.round(kk1,12)==np.round(kk2,12)).all()
amplitude = 1; length_scale=1
K = se_kern(Xtrain, Xtrain, amplitude, length_scale)
K_s = se_kern(Xtrain, Xtest, amplitude, length_scale)
K_ss = se_kern(Xtest, Xtest, amplitude, length_scale)
###Output
_____no_output_____
###Markdown
Samples from the priorWe can sample from prior at test points:$$ f(\mathbf{X}_{\star}) \sim m(\mathbf{X}_{\star}) + \mathbf{L}\mathcal{N}(\boldsymbol0, \mathbf{I}), $$where $k(\mathbf{X}_{\star},\mathbf{X}_{\star}) = \mathbf{L}\mathbf{L}^T$, i.e. $\mathbf{L}$ may be found by the Cholesky decomposition, and $m(\mathbf{X}_{\star})=0$ may be assumed without loss of generality.
###Code
np.random.seed(1)
# Get cholesky decomposition (square root) of the
# covariance matrix
eps=1e-8
L_pri = np.linalg.cholesky(K_ss + eps*np.eye(nX_te)) # add a litte jitter for numerical stability
# Sample 3 sets of standard normals for our test points,
# multiply them by the square root of the covariance matrix
n_samp_pri = 3
f_pri = np.dot(L_pri, np.random.normal(size=(nX_te,n_samp_pri)))
# Now let's plot the 3 sampled functions.
plt.plot(Xtest, f_pri)
plt.plot(Xtrain, ytrain, 'bs', ms=6);
plt.plot(Xtest, ytest, 'gs', ms=3);
plt.xlabel('x');
plt.ylabel('y');
plt.axis([-5, 5, -3, 3])
plt.title('Samples from the GP prior along with the training points');
# plt.show()
###Output
_____no_output_____
###Markdown
Samples from the posteriorIf we condition on the training data, we can also sample from the posterior, as explained in the introduction. We further show the 95\% Bayesian credible interval of the posterior model.
###Code
# Compute posterior
def compute_pos1(Xtr, ytr, Xte, sig_y, sig_f=1.0, l_f=1.0, n_samp_pos=3):
nX_tr = Xtr.shape[0]
nX_te = Xte.shape[0]
eps=1e-8
# Evaluate kernel
K = se_kern(Xtr, Xtr, sig_f, l_f)
K_s = se_kern(Xtr, Xte, sig_f, l_f)
K_ss = se_kern(Xte, Xte, sig_f, l_f)
# Apply Cholesky decomposition
Ly = np.linalg.cholesky(K + np.eye(nX_tr)*std_y**2)
# inverse of covariance
invLy = np.linalg.inv(Ly)
invKy = invLy.T.dot(invLy) # invK = invL^T invL
# alpha
alp_y = invKy.dot(ytr)
# predictive mean
mu_pos = np.dot(K_s.T, alp_y)
# predictive variance
vv_m = np.linalg.solve(Ly,K_s)
cov_pos = K_ss - vv_m.T.dot(vv_m)
std_pos = (np.diag(cov_pos)**(0.5)).reshape(-1,1) # may need abs as variance is zero at training points (no error), which may be encoded as -0 (no allowed)
# Draw samples from the posterior at our test points.
L_pos = np.linalg.cholesky(cov_pos + eps*np.eye(nX_te))
f_pos = mu_pos + np.dot(L_pos, np.random.normal(size=(nX_te,n_samp_pos)))
return(mu_pos, std_pos, f_pos)
# plotting function
def plot_pos1(Xtr, ytr, Xte, yte, f_samps, mu_pos, std_pos):
plt.plot(Xtr, ytr, 'bs', ms=6)
plt.plot(Xte, yte, 'gs', ms=3);
plt.plot(Xte, f_samps);
plt.fill_between(x=Xte[:,0], y1=mu_pos[:,0]-2*std_pos[:,0], y2=mu_pos[:,0]+2*std_pos[:,0], alpha=0.1, color='blue');
plt.plot(Xte, mu_pos, 'r--', lw=2)
plt.xlabel('x');
plt.ylabel('f, y');
plt.axis([-5, 5, -3, 3]);
plt.title('Samples from the GP posterior along with the training data');
# plt.show()
np.random.seed(1)
# Evaluate posterior
mu_pos, std_pos, f_pos = compute_pos1(Xtrain, ytrain, Xtest, std_y)
# plot posterior
plot_pos1(Xtrain, ytrain, Xtest, ytest, f_pos, mu_pos, std_pos)
###Output
_____no_output_____
###Markdown
Parameter learning: Maximum likelihood estimation using gradient decentSee Rasmussen and Williams (2006) for a reference on MLE for Gaussian processes, and e.g. Bishop(2006; Sec. 3.1.3) or Theodoridis(2020; Sec. 5.1 - 5.4) for a reference on gradient decent.We will consider how to find a maximum (marginal) likelihood estimate (MLE) of the parameters using gradient decent (GD) - actually we will perform gradient accent as we are maximizing the log likelihood instead of minimizing its negation, as it is common in the optimization literature.The log $\underline{\text{marginal}}$ likelihood of the data is$$ \log p(\mathbf{y}|\mathbf{X}) = \log \mathcal{N}(\boldsymbol0, \mathbf{K}_y) = - \frac{1}{2} \mathbf{y}^T \mathbf{K}_y^{-1} \mathbf{y} - \frac{1}{2} \log | \mathbf{K}_y | - \frac{N}{2} \log 2 \pi = - \frac{1}{2} \mathbf{y}^T \boldsymbol\alpha - \sum_i \log \mathbf{L}_{ii} - \frac{N}{2} \log 2 \pi$$ where $\mathbf{K}_y = k(\mathbf{X},\mathbf{X})$, $\boldsymbol\alpha = \mathbf{K}^{-1} \mathbf{y} = ( (\mathbf{L}^{-1})^T (\mathbf{L}^{-1}) ) \mathbf{y}$, and $\mathbf{K} = \mathbf{L}\mathbf{L}^T$ is the Cholesky decomposition. The likelihood is called marginal, as the process $f$ is marginalized out of the expression, i.e. $p(\mathbf{y}|\mathbf{X}) = \int p(\mathbf{y},\mathbf{f}|\mathbf{X}) d\mathbf{f}$.We will consider two implementations: (i) without re-parametrization, and (ii) with re-parametrization to ensure non-negativity of the kernel parameters through a log-transform. For case (ii) the deviations get a little bit more complicated, as the derivatives should be calculated wrt. e.g. $\theta_1 = \exp(\sigma^2)$, which requires one more pass through the chain rule of differentiation. In case (i) we ignore the non-negativity constraint, as $(-\sigma)^2 = (\sigma)^2$ etc. Thus, parameter space symmetry is explored; as we will see this makes the algorithm less efficient.***C. E. Rasmussen, C. K. Williams, Gaussian processes for machine learning, MIT press, 2006.Bishop, Christopher M. Pattern recognition and machine learning. springer, 2006.Theodoridis, Sergios. Machine learning: a Bayesian and optimization perspective. Academic Press, 2020.***
###Code
def logMargLike(y, alpha, L, N):
LML = - 0.5 * y.T.dot( alpha ) - np.sum( np.log( np.diag(L) ) ) - 0.5 * N * np.log(2*np.pi)
return(LML)
###Output
_____no_output_____
###Markdown
The derivative wrt. a kernel parameter can generally be expressed as$$ \frac{\partial}{\partial \theta_i} \log p(\mathbf{y}|\mathbf{X}) = \frac{1}{2} \mathbf{y}^T \mathbf{K}_y^{-1} \frac{\partial K_y}{\partial \theta_i} \mathbf{K}_y^{-1} \mathbf{y} - \frac{1}{2} \text{tr} \left( \mathbf{K}_y^{-1} \frac{\partial K_y}{\partial \theta_i} \right) = \frac{1}{2} \text{tr} \left( \left( \boldsymbol\alpha\boldsymbol\alpha^T - \mathbf{K}_y^{-1} \right) \frac{\partial K_y}{\partial \theta_i} \right), $$which we note to be independent of kernel choice! The kernel that we will explore in this tutorial is the squared exponential kernel, which gives us the following general form for the output kernel (i.e. including the output noise variance):$$ k_y(\mathbf{x},\mathbf{x}') = \sigma_f^2 \exp\left( - \frac{1}{2} (\mathbf{x}-\mathbf{x}')^T \mathbf{M} (\mathbf{x}-\mathbf{x}') \right) + \sigma_y^2 \delta_{x,x'}, $$where $\delta_{x,x'}$ is a delta function that includes $\sigma_y^2$ is the expression only when $\mathbf{x}=\mathbf{x}'$. In the simples formulation, which we will consider here, the matrix $\mathbf{M}=l^{-2}\mathbf{I}$ is isotropic and one-dimensional, as $\mathbf{x}$ is one-dimensional, and thus the expression simplifies to$$ k_y(x,x') = \sigma_f^2 \exp\left( - \frac{(x-x')^2}{2 l^2} \right) + \sigma_y^2 \delta_{x,x'}, $$where the first part corr. the square exponential kernel and the second part corr. the output noise variance of the output observations $\mathbf{y}$.
###Code
# Note that se_kern() is defined above
def Ky_kern(x, y, sig_y, sig_f, l_f):
K = se_kern(x, y, sig_f, l_f)
Ky = K + np.eye(len(x))*sig_y**2
return(Ky)
###Output
_____no_output_____
###Markdown
Implementation without parameter transformIn this implementation we consider the parameter vector: $\boldsymbol\theta = \{\sigma, \sigma_f, l\} $, thus without parameters transformations to ensure that the parameters are non-negative. For the above expression, the gradients ${\partial K_y}/{\partial \theta_i}$ may now be defined:$$ \frac{\partial K_y}{\partial \sigma_y} = 2 \sigma_y \delta_{x,x'} $$$$ \frac{\partial K_y}{\partial \sigma_f} = 2 \sigma_f \exp\left( - \frac{(x-x')^2}{2l^2} \right) $$$$ \frac{\partial K_y}{\partial l} = \sigma_f^2 \exp\left( - \frac{(x-x')^2}{2 l^2} \right) \left( \frac{(x-x')^2}{l^3} \right), $$where the last expression $\frac{\partial K_y}{\partial l}$ is derived using the chain rule by redefining $K=\sigma_f^2 \exp(g(l))$, thus $$ \frac{\partial K_y}{\partial l} = \frac{\partial K_y}{\partial g} \frac{\partial g}{\partial l} = \sigma_f^2 \exp\left( - \frac{(x-x')^2}{2 l^2} \right) \frac{\partial g}{\partial l}, $$and given that$$ \frac{\partial g}{\partial l} = \frac{\partial}{\partial l} \left( - \frac{(x-x')^2}{2} l^{-2} \right) = \left( (x-x')^2 l^{-3} \right), $$we arrive at the expression for $\frac{\partial K_y}{\partial l}$ stated above*. ****The issue of defining expressions for the gradients of the SE kernel is also discussed in this Stack Exchange post:https://math.stackexchange.com/questions/1030534/gradients-of-marginal-likelihood-of-gaussian-process-with-squared-exponential-co .***First, we define a function to calculate the gradient for a general parameter:$$ \frac{\partial}{\partial \theta_i} \log p(\mathbf{y}|\mathbf{X}) = \frac{1}{2} \text{tr} \left( \left( \boldsymbol\alpha\boldsymbol\alpha^T - \mathbf{K}_y^{-1} \right) \frac{\partial K_y}{\partial \theta_i} \right), $$
###Code
def calculate_gradient(alpha, invKy, dKy):
d_logML = 0.5 * np.trace( (alpha.dot(alpha.T) - invKy).dot( dKy ) )
return(d_logML)
###Output
_____no_output_____
###Markdown
Next, we define functions to compute the derivative matrices:$$ \frac{\partial K_y}{\partial \sigma_y} = 2 \sigma_y \delta_{x,x'} $$
###Code
def derivative_sig_y(nX, sig_y):
dKy = 2*sig_y*np.eye(nX)
return(dKy)
# test implementation - OK
# def derivative_sig_y_numeric(x,y,sig_y,sig_f,l_f):
# e = 1e-4
# sig_y_lo = sig_y - e
# sig_y_hi = sig_y + e
# dKy = ( Ky_kern(x, y, sig_y_hi, sig_f, l_f) - Ky_kern(x, y, sig_y_lo, sig_f, l_f) ) / (2*e)
# return(dKy)
# mm1=(derivative_sig_y(nX_tr, 0.1)); print(np.diag(mm1))
# mm2=(derivative_sig_y_numeric(Xtrain,ytrain,0.1,1,2)); print(np.diag(mm2))
# (np.round(mm1,3)==np.round(mm2,3)).all()
###Output
_____no_output_____
###Markdown
$$ \frac{\partial K_y}{\partial \sigma_f} = 2 \sigma_f \exp\left( - \frac{(x-x')^2}{2l^2} \right) $$
###Code
def derivative_sig_f(x, sig_f, l_f):
sqdist = np.sum(x**2,1).reshape(-1,1) + np.sum(x**2,1) - 2*np.dot(x, x.T) # reshape(-1,1): from one-dim. to two-dim. array.
dKy = 2 * sig_f * np.exp( - sqdist / (2*l_f**2) )
return(dKy)
# # test implementation ~ OK
# def derivative_sig_f2(x, sig_f, l_f):
# n = x.shape[0]
# dKy = np.zeros((n,n))
# for i in range(n):
# for j in range(n):
# dKy[i,j] = 2 * sig_f * np.exp( -0.5 * (x[i]-x[j])**2 / (l_f**2) )
# return(dKy)
# def derivative_sig_f_numeric(x,y,sig_y,sig_f,l_f):
# e = 1e-8
# sig_f_lo = sig_f - e
# sig_f_hi = sig_f + e
# dKy = ( Ky_kern(x, y, sig_y, sig_f_hi, l_f) - Ky_kern(x, y, sig_y, sig_f_lo, l_f) ) / (2*e)
# return(dKy)
# kk1=derivative_sig_f(Xtrain, 1, 2); print(np.diag(kk1))
# kk2=derivative_sig_f_numeric(Xtrain,ytrain, 0.1, 1, 2); print(np.diag(kk2))
# kk3=derivative_sig_f2(Xtrain, 1, 2); print(np.diag(kk3))
# (np.round(kk1,3)==np.round(kk2,3)).all()
###Output
_____no_output_____
###Markdown
$$ \frac{\partial K_y}{\partial l} = \sigma_f^2 \exp\left( - \frac{(x-x')^2}{2 l^2} \right) \left( \frac{(x-x')^2}{l^3} \right), $$
###Code
def derivative_l_f(x, sig_f, l_f):
sqdist = np.sum(x**2,1).reshape(-1,1) + np.sum(x**2,1) - 2*np.dot(x, x.T) # reshape(-1,1): from one-dim. to two-dim. array.
dKy = sig_f**2 * np.exp(- sqdist / (2*l_f**2) ) * ( sqdist / (l_f**3) )
return(dKy)
# # test implementation ~ OK
# def derivative_l_f2(x, sig_f, l_f):
# n = x.shape[0]
# dKy = np.zeros((n,n))
# for i in range(n):
# for j in range(n):
# dKy[i,j] = sig_f**2 * np.exp( -0.5 * (x[i]-x[j])**2 / (l_f**2) ) * ((x[i]-x[j])**2 / (l_f**3))
# return(dKy)
# def derivative_l_f_numeric(x,y,sig_y,sig_f,l_f):
# e = 1e-8
# l_f_lo = l_f - e
# l_f_hi = l_f + e
# dKy = ( Ky_kern(x, y, sig_y, sig_f, l_f_hi) - Ky_kern(x, y, sig_y, sig_f, l_f_lo) ) / (2*e)
# return(dKy)
# kk1=derivative_l_f(Xtrain, 1, 2); print(np.diag(kk1))
# kk2=derivative_l_f_numeric(Xtrain,ytrain, 0.1, 1, 2); print(np.diag(kk2))
# kk3=derivative_l_f2(Xtrain, 1, 2); print(np.diag(kk3))
# (np.round(kk1,3)==np.round(kk3,3)).all()
###Output
_____no_output_____
###Markdown
Initialize the algorithmNow we are ready to test our implementation, but we first have to initialize the algorithm ...
###Code
# initial values for parameters
# sig_y, sig_f, l_f = 0.1, 1, 2
sig_y, sig_f, l_f = 0.05, 2.0, 3.0
# Apply the kernel function to our training points
Ky0 = Ky_kern(Xtrain, Xtrain, sig_y, sig_f, l_f)
Ly0 = np.linalg.cholesky(Ky0)
# inverse of covariance
invLy0 = np.linalg.inv(Ly0)
invKy0 = invLy0.T.dot(invLy0) # invK = invL^T invL
# alpha
alp0 = invKy0.dot(ytrain)
# log marginal likelihood
logM0 = logMargLike(ytrain, alp0, Ly0, nX_tr)
###Output
_____no_output_____
###Markdown
Run the optimizationThis shows how to run the optimization from óne initialization point; in a real application, we would initialize multiple times overdispersed in the plausible range of the variables and pick the best solution, i.e in terms of likelihood score.
###Code
gamma0 = 0.1 # Step size multiplier
precision = 1e-6 # Desired precision of result
max_epochs = 1000 # Maximum number of runs through the training set
for i in range(max_epochs):
gamma = gamma0 * np.exp(-0.1*i) # temporing
if 0<i:
invKy0 = invKy1
alp0 = alp1
logM0 = logM1
# UPDATA PARAMETERS #####################################
# gradient wrt noise standard deviation
dKsy = derivative_sig_y(nX_tr, std_y)
dMsy = calculate_gradient(alp0, invKy0, dKsy)
# gradient wrt signal standard deviation
dKsf = derivative_sig_f(Xtrain, sig_f, l_f)
dMsf = calculate_gradient(alp0, invKy0, dKsf)
# gradient wrt signal standard deviation
dKlf = derivative_l_f(Xtrain, sig_f, l_f)
dMlf = calculate_gradient(alp0, invKy0, dKlf)
# normalize
dMv = np.array([dMsy, dMsf, dMlf])
dMv = dMv/np.sqrt(dMv.dot(dMv)) # normalize to unit length (stabalizes the algorithm wrt numerical overfolw)
# Change parameters
sig_y += gamma * dMv[0] + eps
sig_f += gamma * dMv[1] + eps
l_f += gamma * dMv[1] + eps
# DEFINE NEW QUANTITIES AND CALCULATE LOG MARG LIKE##########
# Kernel and decomposition
Ky1 = Ky_kern(Xtrain, Xtrain, sig_y, sig_f, l_f)
Ly1 = np.linalg.cholesky(Ky1)
# inverse of covariance
invLy1 = np.linalg.inv(Ly1)
invKy1 = invLy1.T.dot(invLy1) # invK = invL^T invL
# alpha
alp1 = invKy1.dot(ytrain)
# log marginal likelihood
logM1 = logMargLike(ytrain, alp1, Ly1, nX_tr)
print(logM1)
if abs( logM1 - logM0 ) <= abs( logM0 ) * precision:
break
print("Maximum at ", np.array([ sig_y, sig_f, l_f ]), "based on", i, "iterations")
print("Log marginal likelihood:", logM1)
###Output
[[2.77013411]]
[[-0.9608564]]
[[3.20527886]]
[[1.59746382]]
[[3.53671006]]
[[2.88814746]]
[[3.78508996]]
[[3.58241088]]
[[3.96872289]]
[[3.97012256]]
[[4.10310872]]
[[4.19054918]]
[[4.20091273]]
[[4.31579337]]
[[4.27219401]]
[[4.38530175]]
[[4.32471267]]
[[4.421622]]
[[4.36409953]]
[[4.43818405]]
[[4.39373476]]
[[4.44340299]]
[[4.41480219]]
[[4.44286338]]
[[4.42762137]]
[[4.44016996]]
[[4.43335194]]
[[4.43706262]]
[[4.43400861]]
[[4.43377302]]
[[4.43162351]]
[[4.42988878]]
[[4.42763276]]
[[4.42527445]]
[[4.4228012]]
[[4.42026544]]
[[4.41783724]]
[[4.41556455]]
[[4.41350051]]
[[4.41163065]]
[[4.40993906]]
[[4.4084086]]
[[4.4070239]]
[[4.40577107]]
[[4.40463755]]
[[4.40361197]]
[[4.40268404]]
[[4.40184446]]
[[4.40108481]]
[[4.40039748]]
[[4.39977557]]
[[4.39921287]]
[[4.39870374]]
[[4.39824306]]
[[4.39782623]]
[[4.39744907]]
[[4.39710781]]
[[4.39679903]]
[[4.39651963]]
[[4.39626683]]
[[4.39603808]]
[[4.39583109]]
[[4.39564381]]
[[4.39547434]]
[[4.395321]]
[[4.39518225]]
[[4.3950567]]
[[4.3949431]]
[[4.3948403]]
[[4.39474729]]
[[4.39466312]]
[[4.39458696]]
[[4.39451804]]
[[4.39445568]]
[[4.39439925]]
[[4.39434819]]
[[4.39430198]]
[[4.39426017]]
[[4.39422233]]
[[4.39418809]]
[[4.3941571]]
[[4.39412906]]
[[4.39410369]]
[[4.39408072]]
[[4.39405994]]
[[4.39404113]]
[[4.39402411]]
[[4.3940087]]
[[4.39399476]]
[[4.39398214]]
[[4.39397072]]
[[4.39396038]]
[[4.39395102]]
[[4.39394255]]
[[4.39393488]]
[[4.39392794]]
[[4.39392166]]
[[4.39391597]]
[[4.39391081]]
[[4.39390615]]
[[4.39390192]]
Maximum at [0.10234626 2.04212893 3.04212893] based on 100 iterations
Log marginal likelihood: [[4.39390192]]
###Markdown
Plot the resulting modelSample from the posterior and 95\% Bayesian credible interval of the posterior model.
###Code
np.random.seed(1)
# Evaluate posterior
mu_pos, std_pos, f_pos = compute_pos1(Xtrain, ytrain, Xtest, sig_y, sig_f, l_f)
# plot posterior
plot_pos1(Xtrain, ytrain, Xtest, ytest, f_pos, mu_pos, std_pos)
###Output
_____no_output_____
###Markdown
Implementation with parameter transformIn this implementation, we define the parameter vector: $\boldsymbol\theta = \{\log \sigma^2, \log \sigma_f^2, \log l^2\} $, thus we transform the parameters to ensure that the parameters are non-negative. For the above expression, the gradients ${\partial K_y}/{\partial \theta_i}$ may now be defined:$$ \frac{\partial K_y}{\partial \theta_1} = \exp(\theta_1) \delta_{x,x'} $$$$ \frac{\partial K_y}{\partial \theta_2} = \exp(\theta_2) \exp\left( - \frac{(x-x')^2}{2 \exp(\theta_3)} \right) $$$$ \frac{\partial K_y}{\theta_3} = \exp(\theta_2) \exp\left( - \frac{(x-x')^2}{2 \exp(\theta_3)} \right) \left( \frac{(x-x')^2}{2 \exp(\theta_3)} \right), $$as $K_y$ i the re-parameterized form reads:$$ K_y(x,x') = \exp(\theta_2) \exp\left( - \frac{(x-x')^2}{2 \exp(\theta_3)} \right) + \exp(\theta_1) \delta_{x,x'}, $$
###Code
# theta = log sigma_y
def derivative_sig_y_theta1(nX, sig_y):
dKy = (sig_y**2)*np.eye(nX)
return(dKy)
# theta = log sigma_f
def derivative_sig_f_theta2(x, sig_f, l_f):
sqdist = np.sum(x**2,1).reshape(-1,1) + np.sum(x**2,1) - 2*np.dot(x, x.T) # reshape(-1,1): from one-dim. to two-dim. array.
dKy = (sig_f**2) * np.exp(- sqdist / ( 2 * l_f**2 ) )
return(dKy)
# theta = log l_f
def derivative_l_f_theta3(x, sig_f, l_f):
sqdist = np.sum(x**2,1).reshape(-1,1) + np.sum(x**2,1) - 2*np.dot(x, x.T) # reshape(-1,1): from one-dim. to two-dim. array.
dKy = (sig_f**2) * np.exp(- sqdist / (2*l_f**2) ) * ( sqdist / (2 * l_f**2) )
return(dKy)
###Output
_____no_output_____
###Markdown
Initialize the algorithmNow we are ready to test our implementation, but we first have to initialize the algorithm ...
###Code
# initial values for parameters
sig_y = 0.05; theta1 = np.log(sig_y**2)
sig_f = 2; theta2 = np.log(sig_f**2)
l_f = 3; theta3 = np.log(l_f**2)
# Apply the kernel function to our training points
Ky0 = Ky_kern(Xtrain, Xtrain, sig_y, sig_f, l_f)
Ly0 = np.linalg.cholesky(Ky0)
# inverse of covariance
invLy0 = np.linalg.inv(Ly0)
invKy0 = invLy0.T.dot(invLy0) # invK = invL^T invL
# alpha
alp0 = invKy0.dot(ytrain)
# log marginal likelihood
logM0 = logMargLike(ytrain, alp0, Ly0, nX_tr)
# logM0 = -1e16
###Output
_____no_output_____
###Markdown
Run the optimizationThis again shows how to run the optimization from óne initialization point; in a real application, we would initialize multiple times overdispersed in the plausible range of the variables and pick the best solution, i.e in terms of likelihood score.
###Code
gamma0 = 1 # Step size multiplier
precision = 1e-8 # Desired precision of result
max_epochs = 1000 # Maximum number of runs through the training set
for i in range(max_epochs):
gamma = gamma0 * np.exp(-0.9*i) # temporing
if 0<i:
invKy0 = invKy1
alp0 = alp1
logM0 = logM1
# UPDATA PARAMETERS #####################################
# gradient wrt theta1 (noise standard deviation)
dKsy = derivative_sig_y_theta1(nX_tr, std_y)
dMsy = calculate_gradient(alp0, invKy0, dKsy)
# gradient wrt theta2 (signal standard deviation)
dKsf = derivative_sig_f_theta2(Xtrain, sig_f, l_f)
dMsf = calculate_gradient(alp0, invKy0, dKsf)
# gradient wrt theta3 (signal length scale)
dKlf = derivative_l_f_theta3(Xtrain, sig_f, l_f)
dMlf = calculate_gradient(alp0, invKy0, dKlf)
# Change parameters
dMtheta = np.array([dMsy, dMsf, dMlf])
dMtheta = dMtheta/np.sqrt(dMtheta.dot(dMtheta)) # normalize to unit length (stabalizes the algorithm wrt numerical overfolw)
theta1 += gamma * dMtheta[0]
theta2 += gamma * dMtheta[1]
theta3 += gamma * dMtheta[2]
sig_y = np.sqrt( np.exp(theta1) ) + eps
sig_f = np.sqrt( np.exp(theta2) ) + eps
l_f = np.sqrt( np.exp(theta3) ) + eps
# DEFINE NEW QUANTITIES AND CALCULATE LOG MARG LIKE##########
# Kernel and decomposition
Ky1 = Ky_kern(Xtrain, Xtrain, sig_y, sig_f, l_f)
Ly1 = np.linalg.cholesky(Ky1)
# inverse of covariance
invLy1 = np.linalg.inv(Ly1)
invKy1 = invLy1.T.dot(invLy1) # invK = invL^T invL
# alpha
alp1 = invKy1.dot(ytrain)
# log marginal likelihood
logM1 = logMargLike(ytrain, alp1, Ly1, nX_tr) # without extre regularization (not needed for small dimensiaonal problems, Rasmussen&Williams(2006))
print(logM1)
if abs( logM1 - logM0 ) <= abs( logM0 ) * precision:
break
print("Maximum at ", np.array([ sig_y, sig_f, l_f ]), "based on", i, "iterations")
###Output
[[5.84187491]]
[[6.77280165]]
[[6.92041437]]
[[6.98295995]]
[[7.00740403]]
[[7.01722184]]
[[7.02119383]]
[[7.02280548]]
[[7.02346019]]
[[7.02372629]]
[[7.02383447]]
[[7.02387844]]
[[7.02389632]]
[[7.02390359]]
[[7.02390655]]
[[7.02390775]]
[[7.02390824]]
[[7.02390844]]
[[7.02390852]]
[[7.02390855]]
Maximum at [0.09036608 1.81758084 2.20874715] based on 19 iterations
###Markdown
Plot the resulting modelSample from the posterior and 95\% Bayesian credible interval of the posterior model.
###Code
np.random.seed(1)
# Evaluate posterior
mu_pos, std_pos, f_pos = compute_pos1(Xtrain, ytrain, Xtest, sig_y, sig_f, l_f)
# plot posterior
plot_pos1(Xtrain, ytrain, Xtest, ytest, f_pos, mu_pos, std_pos)
###Output
_____no_output_____
###Markdown
Parameter learning: Metropolis and Metropolis-Hastings algorithmSee e.g. Gelman et al (2013; ch.11-12) for a reference on the Metropolis and Metropolis-Hastings algorithm.In this section, we implement the Metropolis and Metropolis-Hastings algorithm, i.e. one implementation considers a symmetric sampling distribution in log-space, and the other use an asymmetric jumping kernel, which is implemented as a Gaussian copula. To consider the one or the other you simply have to uncomment the implementation you want to study and comment out the other one. The generative storyThe generative model reads;$$ p(\theta |\mathcal{D}) \propto p(\mathbf{y}|\mathbf{X},\boldsymbol\theta) p(\boldsymbol\theta), $$where $$ {\theta_i} = \text{Inv-Gamma}(a,b), $$and $\{\theta_i\}_{i=1}^3 = \{ \sigma^2, \sigma_f^2, l^2 \}$. The hyper-parameters $a$ and $b$ are pre-set in this implementation but could also be learnt. The unnormalized parameter posteriorRecall that the parameter posterior is defined through Bayes' rule as $$p(\boldsymbol\theta|\mathcal{D}) = \frac{ p(\mathbf{y},\boldsymbol\theta|\mathbf{x}) }{ p(\mathbf{y})}, $$but we will work with the unnormalized version, i.e.$$p(\boldsymbol\theta|\mathcal{D}) \propto p(\mathbf{y},\boldsymbol\theta|\mathbf{x}), $$to avoid calculating the always troubling normalizing constant $p(\mathbf{y})$.***Gelman, Andrew, et al. Bayesian data analysis. CRC press, 2013.***
###Code
# This function calculates the unnormalized posterior for theta, i.e. p(theta|D) ~ p(y,theta|x)
def log_pos_metropolis(x,y,theta,pam1,pam2,pam3):
N = x.shape[0]
sig_y = np.sqrt(theta[0])
sig_f = np.sqrt(theta[1])
l_f = np.sqrt(theta[2])
# Apply the kernel function to our training points
Ky = Ky_kern(x, x, sig_y, sig_f, l_f)
Ly = np.linalg.cholesky(Ky)
# inverse of covariance
invLy = np.linalg.inv(Ly)
invKy = invLy.T.dot(invLy) # invK = invL^T invL
# alpha
alp = invKy.dot(y)
# log marginal posterior
logPos = (logMargLike(y, alp, Ly, N)
+ ss.invgamma(a = pam1[0], scale = pam1[1]).logpdf(theta[0])
+ ss.invgamma(a = pam2[0], scale = pam2[1]).logpdf(theta[1])
+ ss.invgamma(a = pam3[0], scale = pam3[1]).logpdf(theta[2])
)
return(logPos)
# log_pos_metropolis(Xtrain, ytrain, np.array([std_y**2, 1**2, 0.1**2]), np.array([3,1]), np.array([3,1]), np.array([3,1]))
# Make sure we are using the scikit implementation correctly
# https://en.wikipedia.org/wiki/Inverse-gamma_distribution
def my_invgamma_pdf(x,a,b):
gpdf = np.zeros(len(x))
ind = (x>0)
gpdf[ind] = ( (b**a)/math.gamma(a) ) * (1/x[ind])**(a+1) * np.exp( -b/x[ind] )
return(gpdf)
xx=np.arange(-0.5,5,0.01)
yy1=ss.invgamma.pdf(xx, a=2, scale=2)
yy2=my_invgamma_pdf(xx, 2, 2)
plt.figure(figsize=(5, 3))
plt.subplot(1, 2, 1)
plt.plot(xx,yy1)
plt.xlabel('x')
plt.ylabel('pdf')
plt.title('Scikit function')
plt.subplot(1, 2, 2)
plt.plot(xx,yy2)
plt.title('My implementation')
plt.xlabel('x')
plt.tight_layout()
###Output
_____no_output_____
###Markdown
Metropolis algorithmNote that we sample $\log \boldsymbol\theta$ using a Gaussian jumping distribution in this implementation. Sampling from the posteriorThis shows how to sample from éne chain; in a real application, we would initialize multiple chains overdispersed in the plausible range of the variables and check for mixing.
###Code
# # sigma is assigned an informative prior to keep samples at a realonable level
# pam1 = np.array([2,1/8]); pam2 = np.array([2,2]); pam3 = np.array([2,2]) # choose by CV
# # pam1 = np.array([2,2]); pam2 = np.array([2,2]); pam3 = np.array([2,2])
# np.random.seed(100)
# n_samp_met = 10**3
# eps_met = 1e-8
# # np.warnings.filterwarnings('error', category=np.VisibleDeprecationWarning)
# # METROPOLIS ALGORITHM
# samp_met = np.zeros([n_samp_met, 3])
# samp_met[0,:] = np.log(np.array([0.01, 1., 2.])**2)
# lp_cur_met = log_pos_metropolis(Xtrain, ytrain, np.exp(samp_met[0,:]), pam1, pam2, pam3)
# proposal_stds = np.abs(samp_met[0,:])*0.05
# counter=0
# for t in range(1,n_samp_met,1):
# x_pro_met = ss.multivariate_normal( mean = samp_met[t-1,:], cov = (np.diag(proposal_stds) + eps_met) ).rvs(1)
# lp_pro_met = log_pos_metropolis(Xtrain, ytrain, np.exp(x_pro_met), pam1, pam2, pam3)
# ratio_pro_met = np.min( [(lp_pro_met - lp_cur_met )[0,0], 0] )
# if np.log(np.random.uniform()) < ratio_pro_met:
# counter += 1
# samp_met[t,:] = x_pro_met
# lp_cur_met = lp_pro_met
# else:
# samp_met[t,:] = samp_met[t-1,:]
# samp_met = np.exp(samp_met) # transform back to the scale of sig_y, sig_f, and l_f
# print('Acceptance ratio: ', counter/n_samp_met)
###Output
_____no_output_____
###Markdown
Metropolis-Hastings algorithmNote that we sample from a set of log-normal distributions via a Gaussian copula implementation (see below). Proposal distribution for Metropolis-Hastings algorithm: Gaussian copula representation of log-normalThis is a little overkill for this example, but it builds the foundation for the construction of more complicated jumping distributions, e.g. including covariance information.
###Code
def logNorm2Norm_parameters(lm ,ls):
phi = np.sqrt( ls**2 + lm**2 )
nm = 2 * np.log(lm) - np.log(phi) # mean of log(Y)
ns = np.sqrt( 2*np.log(phi) - 2*np.log(lm) ) # std dev of log(Y)
return(nm,ns)
# This implementation corresponds to a Gaussian copula, see e.g.
# https://se.mathworks.com/help/stats/copulas-generate-correlated-samples.html
# https://www.sciencedirect.com/science/article/abs/pii/0266892086900330?via%3Dihub
def proposal_distribution(mu_v,sigma_v):
m = len(mu_v)
MVN = ss.multivariate_normal( mean = np.zeros(m), cov = np.eye(m) )
Z = MVN.rvs(1) # define a correlation if needed
logprobZ = MVN.logpdf(Z) # probability of realization
U = ss.norm.cdf(Z)
X = np.zeros(U.shape)
for i in range(m):
nm, ns = logNorm2Norm_parameters(mu_v[i] ,sigma_v[i])
X[i] = ss.lognorm(s=ns, scale=np.exp(nm)).ppf(U[i]) # define transformation 1
return(X,logprobZ)
def inv_proposal_distribution(mu_v,sigma_v,x):
m = len(mu_v)
U = np.zeros(x.shape)
for i in range(m):
nm, ns = logNorm2Norm_parameters(mu_v[i] ,sigma_v[i])
U[i] = ss.lognorm(s=ns, scale=np.exp(nm)).cdf(x[i]) # define transformation 1
Z = ss.norm.ppf(U)
logprob = ss.multivariate_normal( mean = np.zeros(m), cov = np.eye(m) ).logpdf(Z)
return(logprob)
# xx, llpp = proposal_distribution(np.array([0.1,0.1,0.1]), np.array([1,1,1])); print(xx, llpp)
# llpp2 = inv_proposal_distribution(np.array([0.1,0.1,0.1]), np.array([1,1,1]),xx); print(llpp2)
# uu = np.random.uniform(size=1000000)
# nnm,nns = logNorm2Norm_parameters(10 ,10)
# xx=ss.lognorm(s=nns, scale=np.exp(nnm)).ppf(uu)
###Output
_____no_output_____
###Markdown
Sampling from the posteriorThis shows how to sample from éne chain; in a real application, we would initialize multiple chains overdispersed in the plausible range of the variables and check for mixing.
###Code
# sigma is assigned an informative prior to keep samples at a realonable level
pam1 = np.array([2,1/8]); pam2 = np.array([2,2]); pam3 = np.array([2,2]) # choose by CV
# pam1 = np.array([2,2]); pam2 = np.array([2,2]); pam3 = np.array([2,2])
np.random.seed(100)
n_samp_met = 10**3
eps_met = 1e-8
# np.warnings.filterwarnings('error', category=np.VisibleDeprecationWarning)
# METROPOLIS-HASTINGS ALGORITHM
samp_met = np.zeros([n_samp_met, 3])
samp_met[0,:] = np.array([0.1, 1., 2.])**2 + eps
lp_cur_met = log_pos_metropolis(Xtrain, ytrain, samp_met[0,:], pam1, pam2, pam3)
proposal_stds = samp_met[0,:]*0.15 + eps
counter=0
for t in range(1,n_samp_met,1):
x_pro_met, logprob_pro_met = proposal_distribution( samp_met[t-1,:] , proposal_stds )
lp_pro_met = log_pos_metropolis(Xtrain, ytrain, x_pro_met, pam1, pam2, pam3)
logprob_cur_met = inv_proposal_distribution( x_pro_met, proposal_stds , samp_met[t-1,:] )
ratio_pro_met = np.min( [(lp_pro_met - lp_cur_met + logprob_cur_met - logprob_pro_met)[0,0], 0]) # log-space formulation
if np.log(np.random.uniform()) < ratio_pro_met:
counter += 1
samp_met[t,:] = x_pro_met
lp_cur_met = lp_pro_met
else:
samp_met[t,:] = samp_met[t-1,:]
print('Acceptance ratio: ', counter/n_samp_met)
###Output
Acceptance ratio: 0.435
###Markdown
Analyze samplesWe regard the first half of the samples as burn-in, and plot the burn-in samples (red) along with the posterior samples (blue).
###Code
samp_met0 = samp_met[0:round(n_samp_met/2),:] # burn-in
samp_met1 = samp_met[round(n_samp_met/2):n_samp_met,:] # without burn-in
plt.figure()
plt.hist(np.sqrt( samp_met0[:,0]), color='red' );
plt.hist(np.sqrt( samp_met1[:,0]) , color='blue' );
plt.title('Noise standard deviation')
plt.figure()
plt.hist(np.sqrt( samp_met0[:,1]), color='red' );
plt.hist(np.sqrt( samp_met1[:,1]) , color='blue' );
plt.title('Kernel standard deviation (signal)')
plt.figure()
plt.hist(np.sqrt( samp_met0[:,2]), color='red' );
plt.hist(np.sqrt( samp_met1[:,2]) , color='blue' );
plt.title('Kernel length scale (signal)');
###Output
_____no_output_____
###Markdown
Posterior predictive distribution: Point estimateHere we pick óne parameter setting for the parameters. Plot the resulting modelSample from the posterior and 95\% Bayesian credible interval of the posterior model.
###Code
np.random.seed(1)
par_est = np.sqrt(ss.mode(samp_met1)[0][0]); print('Parameter estimates: ', par_est)
# par_est = np.median(samp_met1, axis=0); print(par_est)
# par_est = np.sqrt(np.mean(samp_met1,axis=0)); print(par_est)
# Evaluate posterior
mu_pos, std_pos, f_pos = compute_pos1(Xtrain, ytrain, Xtest, par_est[0], par_est[1], par_est[2])
# plot posterior
plot_pos1(Xtrain, ytrain, Xtest, ytest, f_pos, mu_pos, std_pos)
###Output
Parameter estimates: [0.12403367 0.51203448 0.83233201]
###Markdown
Posterior predictive distribution: Full Bayesian inferenceHere we average over the posterior samples. Evaluate mean function and covariance for all posterior samples
###Code
n_samp = samp_met1.shape[0]
m_points = Xtest.shape[0]
mu_pos_samples = np.zeros(( n_samp, m_points ))
std_pos_samples = np.zeros(( n_samp, m_points ))
for i in range(n_samp):
par_vec_i = np.sqrt(samp_met1[i,:])
amplitude = par_vec_i[1]; length_scale=par_vec_i[2]
K = se_kern(Xtrain, Xtrain, amplitude, length_scale)
K_s = se_kern(Xtrain, Xtest, amplitude, length_scale)
K_ss = se_kern(Xtest, Xtest, amplitude, length_scale)
# Apply the kernel function to our training points
L = np.linalg.cholesky(K + np.eye(nX_tr)*par_vec_i[0]**2)
# predictive mean
alp_m = np.linalg.solve(L.T,np.linalg.solve(L, ytrain))
mu_pos = np.dot(K_s.T, alp_m)
# predictive variance
vv_m = np.linalg.solve(L,K_s)
cov_pos = K_ss - vv_m.T.dot(vv_m)
std_pos = (np.diag(cov_pos)**(0.5)).reshape(-1,1)
mu_pos_samples[i,:] = mu_pos.T
std_pos_samples[i,:] = std_pos.T
###Output
_____no_output_____
###Markdown
Define sample statistics for the latent function (f) and the output (y)
###Code
# Define sample statistics for the latent function (f) and the output (y)
f_stats = np.zeros((m_points,3))
y_stats = np.zeros((m_points,3))
noise_y = np.random.normal(np.zeros(n_samp))
for i in range(m_points):
f_samp_i = mu_pos_samples[:,i]
f_samp_i_m = np.mean(f_samp_i)
f_samp_i_q = np.quantile(f_samp_i, [0.025, 0.975])
f_stats[i,:] = np.concatenate(([f_samp_i_m],f_samp_i_q), axis=0)
y_samp_i = mu_pos_samples[:,i] + noise_y * std_pos_samples[:,i]
y_samp_i_m = np.mean(y_samp_i)
y_samp_i_q = np.quantile(y_samp_i, [0.025, 0.975])
y_stats[i,:] = np.concatenate(([y_samp_i_m],y_samp_i_q), axis=0)
###Output
_____no_output_____
###Markdown
Plot the resulting model95\% Bayesian credible interval of the posterior model.
###Code
# Plot training and test data
plt.plot(Xtrain, ytrain, 'bs', ms=6)
plt.plot(Xtest, ytest, 'gs', ms=3);
# Predictive mean
plt.plot(Xtest, f_stats[:,0], 'r--', lw=2)
# Shade in credible region
plt.fill_between(Xtest[:,0], y_stats[:,1], y_stats[:,2], alpha=0.1, color='blue')
plt.fill_between(Xtest[:,0], f_stats[:,1], f_stats[:,2], alpha=0.5)
plt.axis([-5, 5, -3, 3]);
plt.xlabel('x');
plt.ylabel('f, y');
plt.title('Samples from the GP posterior along with the training data');
###Output
_____no_output_____
###Markdown
Parameter learning: Hamiltonian Monte Carlo algorithmSee e.g. Gelman et al. (2013; Sec.12.4 and App.C.4 (R implementation)) for a reference on the Hamiltonian Monte Carlo algorithm. The generative storyIn this section, we will use a log-normal proposal distribution (non-symmetric) and the following generative model in the MCMC of Metropolis-Hastings;$$ p(\theta |\mathcal{D}) \propto p(\mathbf{y}|\mathbf{X},\boldsymbol\theta) p(\boldsymbol\theta), $$where $$ {\theta_i}^2 = \text{Inv-Gamma}(a,b), $$and $\{\theta_i\}_{i=1}^3 = \{ \sigma, \sigma_f, l \}$, with the hyper-parameters $a$ and $b$ pre-set in this implementation. Thus, we will parameterize the model as we did when performing MLE above (without parameters transformations), in order to reuse some of the functions already implemented. We could also for this case consider a parameter transformation, and define the probabilistic model in the transformed space using the Jacobien, see Gelman et al.(2013). The unnormalized parameter posteriorRecall that the parameter posterior is defined through Bayes' rule as $$p(\boldsymbol\theta|\mathcal{D}) = \frac{ p(\mathbf{y},\boldsymbol\theta|\mathbf{x}) }{ p(\mathbf{y})}, $$but we will work with the unnormalized version, i.e.$$p(\boldsymbol\theta|\mathcal{D}) \propto p(\mathbf{y},\boldsymbol\theta|\mathbf{x}), $$to avoid calculating the always troubling normalizing constant $\mathbf{y}$.***Gelman, Andrew, et al. Bayesian data analysis. CRC press, 2013.***
###Code
# This function calculates the unnormalized posterior for theta, i.e. p(theta|D) = p(y,theta|x)/p(y)
def log_pos_hmc(x,y,theta,pam1,pam2,pam3):
if (np.any(np.isnan(theta))):
logPos = -1e-16
elif (np.any(theta <= 0)):
logPos = -1e-16
else:
N = x.shape[0]
sig_y = theta[0]
sig_f = theta[1]
l_f = theta[2]
# Apply the kernel function to our training points
Ky = Ky_kern(x, x, sig_y, sig_f, l_f)
Ly = np.linalg.cholesky(Ky)
# inverse of covariance
invLy = np.linalg.inv(Ly)
invKy = invLy.T.dot(invLy) # invK = invL^T invL
# alpha
alp = invKy.dot(y)
# log marginal posterior
logPos = (logMargLike(y, alp, Ly, N)
+ ss.invgamma(a = pam1[0], scale = pam1[1]).logpdf(theta[0]**2)
+ ss.invgamma(a = pam2[0], scale = pam2[1]).logpdf(theta[1]**2)
+ ss.invgamma(a = pam3[0], scale = pam3[1]).logpdf(theta[2]**2)
)
return(logPos)
# log_pos_hmc(Xtrain, ytrain, np.array([std_y, 1, 2]), np.array([2,2]), np.array([2,2]), np.array([2,2]))
# log_pos_hmc(Xtrain, ytrain, np.array([std_y, np.nan, 2]), np.array([2,2]), np.array([2,2]), np.array([2,2]))
# log_pos_hmc(Xtrain, ytrain, np.array([std_y, -1, 2]), np.array([2,2]), np.array([2,2]), np.array([2,2]))
###Output
_____no_output_____
###Markdown
Gradients of the posterior modelAs note we can re-use the gradients already derived for the likelihood part, so we simply have to define the gradients for the inverse gamma terms and combine the likelihood and prior terms. Gradients of inverse gamma
###Code
def my_invgamma_gradient_x(x,a,b):
grad = -2*(a+1) / x + 2 * b * x**(-3)
return(grad)
# Test implementation
# def my_invgamma_gradient_numerical(x,a,b):
# e = 1e-4
# x_lo = x**2 - e
# x_hi = x**2 + e
# grad = ( np.log(my_invgamma_pdf(x_hi, a, b) ) - np.log( my_invgamma_pdf(x_lo, a, b) ) ) / (2*e)
# return(grad)
#
# # Test
# xtt = np.array([0.5])
# print( my_invgamma_gradient_x2(xtt,2,2) )
# print( my_invgamma_gradient_numerical(xtt,2,2) )
###Output
_____no_output_____
###Markdown
Gradients of parameter posterior
###Code
def hmc_gradients(x,y,theta,pam1,pam2,pam3):
nX = x.shape[0]
Ky = Ky_kern(x, x, theta[0], theta[1], theta[2]) + np.eye(nX)*1e-8
Ly = np.linalg.cholesky(Ky)
invLy = np.linalg.inv(Ly)
invKy = invLy.T.dot(invLy) # invK = invL^T invL
alp = invKy.dot(y)
# gradient wrt noise standard deviation
dKsy = derivative_sig_y(nX, theta[0])
dMsy = calculate_gradient(alp, invKy, dKsy) + my_invgamma_gradient_x(theta[0],pam1[0],pam1[1])
# gradient wrt signal standard deviation
dKsf = derivative_sig_f(x, theta[1], theta[2])
dMsf = calculate_gradient(alp, invKy, dKsf) + my_invgamma_gradient_x(theta[1],pam2[0],pam2[1])
# gradient wrt signal standard deviation
dKlf = derivative_l_f(x, theta[1], theta[2])
dMlf = calculate_gradient(alp, invKy, dKlf) + my_invgamma_gradient_x(theta[2],pam3[0],pam3[1])
dMtheta = np.array([dMsy, dMsf, dMlf])
return(dMtheta)
# Test implementation - OK
def hmc_gradients_numeric(x,y,theta,pam1,pam2,pam3):
d = len(theta)
e = 1e-4
grad = np.zeros(d)
for i in range(d):
th_lo = theta.copy();
th_hi = theta.copy();
th_lo[i] -= e;
th_hi[i] += e;
grad[i] = ( log_pos_hmc(x,y,th_hi,pam1,pam2,pam3) - log_pos_hmc(x,y,th_lo,pam1,pam2,pam3) ) / (2*e)
return(grad)
print(hmc_gradients(Xtrain, ytrain, np.array([0.1, 1., 2.]), np.array([2,2]), np.array([2,2]), np.array([2,2])))
print(hmc_gradients_numeric(Xtrain, ytrain, np.array([0.1, 1., 2.]), np.array([2,2]), np.array([2,2]), np.array([2,2])))
###Output
[ 3.91239156e+03 9.04441012e-02 -4.83252533e+00]
[ 3.91239984e+03 9.04443554e-02 -4.83252563e+00]
###Markdown
Sampling from the posteriorThis shows how to sample from éne chain; in a real application, we would initialize multiple chains overdispersed in the plausible range of the variables and check for mixing. Function implementing óne HMC iteration
###Code
# One HMC iteration
def hmc_iteration(x,y,theta,pam1,pam2,pam3,epsilon,nLeap,M):
M_inv = 1/M
d = len(theta)
# initialize
phi = np.random.normal(loc=np.zeros(d), scale=np.sqrt(M))
theta0 = theta.copy()
# log posterior
log_pos0 = log_pos_hmc(x,y,theta,pam1,pam2,pam3) - 0.5*np.sum(M_inv*phi**2)
# leapfrog steps
phi += 0.5*epsilon*hmc_gradients(x,y,theta,pam1,pam2,pam3)
for l in range(nLeap):
theta += epsilon*M_inv*phi
if (l==(nLeap-1)):
phi += 0.5*epsilon*hmc_gradients(x,y,theta,pam1,pam2,pam3)
else:
phi += 1.0*epsilon*hmc_gradients(x,y,theta,pam1,pam2,pam3)
# Metropolis step
log_pos1 = log_pos_hmc(x,y,theta,pam1,pam2,pam3) - 0.5*np.sum(M_inv*phi**2)
ratio = np.min( [(log_pos1 - log_pos0 )[0,0], 0] )
if np.log(np.random.uniform()) < ratio:
theta1 = theta
counter = 1
else:
theta1 = theta0
counter = 0
return(theta1, counter)
# hmc_iteration(Xtrain,ytrain,np.array([0.1, 1., 2.]),np.array([2,2]),np.array([2,2]),np.array([2,2]),0.001, 3, np.ones(3))
###Output
_____no_output_____
###Markdown
Sampling from the posterior - one chain wrapper for the function above
###Code
# np.warnings.filterwarnings('error', category=np.VisibleDeprecationWarning)
np.random.seed(100)
n_samp_hmc = 10**3
epsilon0 = 0.1
nLeap0 = 10
eps = 1e-8
samp_hmc = np.zeros([n_samp_hmc, 3])
samp_hmc[0,:] = np.array([0.1, 1., 2.]) + eps
mass_vec = 1/(2*samp_hmc[0,:]**2) + eps
counter = 0
for t in range(1,n_samp_hmc,1):
th_t, count_t = hmc_iteration(Xtrain, ytrain,
samp_hmc[t-1,:],
np.array([2,1/8]), np.array([2,2]), np.array([2,2]),
epsilon0, nLeap0 , mass_vec)
samp_hmc[t,:] = th_t.copy()
counter += count_t
print('Acceptance rate: ', counter/n_samp_hmc) # Gelman (2013) recommend ~60-70% for HMC
###Output
Acceptance rate: 0.633
###Markdown
Analyze samplesWe regard the first half of the samples as burn-in and plot the posterior samples (blue).
###Code
samp_hmc0 = samp_hmc[0:round(n_samp_hmc/2),:] # burn-in
samp_hmc1 = samp_hmc[round(n_samp_hmc/2):n_samp_hmc,:] # without burn-in
plt.figure()
# plt.hist(samp_hmc0[:,0], color='red' );
plt.hist(samp_hmc1[:,0] , color='blue' );
plt.title('Noise standard deviation')
plt.figure()
# plt.hist(samp_hmc0[:,1], color='red' );
plt.hist(samp_hmc1[:,1] , color='blue' );
plt.title('Kernel standard deviation (signal)')
plt.figure()
# plt.hist(samp_hmc0[:,2], color='red' );
plt.hist(samp_hmc1[:,2] , color='blue' );
plt.title('Kernel length scale (signal)');
###Output
_____no_output_____
###Markdown
Posterior predictive distribution: Point estimateHere we pick óne parameter setting for the parameters. Plot the resulting modelSample from the posterior and 95\% Bayesian credible interval of the posterior model.
###Code
np.random.seed(1)
# Parameter estimates
par_est = (ss.mode(samp_hmc1)[0][0]); print('Parameter estimates ', par_est)
# Evaluate posterior
mu_pos, std_pos, f_pos = compute_pos1(Xtrain, ytrain, Xtest, par_est[0], par_est[1], par_est[2])
# plot posterior
plot_pos1(Xtrain, ytrain, Xtest, ytest, f_pos, mu_pos, std_pos)
###Output
Parameter estimates [0.14672252 0.77689273 1.76872226]
###Markdown
Posterior predictive distribution: Full Bayesian inferenceHere we average over the posterior samples. Evaluate mean function and covariance for all posterior samples
###Code
n_samp = samp_hmc1.shape[0]
m_points = Xtest.shape[0]
mu_pos_samples = np.zeros(( n_samp, m_points ))
std_pos_samples = np.zeros(( n_samp, m_points ))
for i in range(n_samp):
par_vec_i = samp_hmc1[i,:]
amplitude = par_vec_i[1]; length_scale=par_vec_i[2]
K = se_kern(Xtrain, Xtrain, amplitude, length_scale)
K_s = se_kern(Xtrain, Xtest, amplitude, length_scale)
K_ss = se_kern(Xtest, Xtest, amplitude, length_scale)
# Apply the kernel function to our training points
L = np.linalg.cholesky(K + np.eye(nX_tr)*par_vec_i[0]**2)
# predictive mean
alp_m = np.linalg.solve(L.T,np.linalg.solve(L, ytrain))
mu_pos = np.dot(K_s.T, alp_m)
# predictive variance
vv_m = np.linalg.solve(L,K_s)
cov_pos = K_ss - vv_m.T.dot(vv_m)
std_pos = (np.diag(cov_pos)**(0.5)).reshape(-1,1)
mu_pos_samples[i,:] = mu_pos.T
std_pos_samples[i,:] = std_pos.T
###Output
_____no_output_____
###Markdown
Define sample statistics for the latent function (f) and the output (y)
###Code
f_stats = np.zeros((m_points,3))
y_stats = np.zeros((m_points,3))
noise_y = np.random.normal(np.zeros(n_samp))
for i in range(m_points):
f_samp_i = mu_pos_samples[:,i]
f_samp_i_m = np.mean(f_samp_i)
# f_samp_i_m = np.quantile(f_samp_i, 0.5)
f_samp_i_q = np.quantile(f_samp_i, [0.025, 0.975])
f_stats[i,:] = np.concatenate(([f_samp_i_m],f_samp_i_q), axis=0)
y_samp_i = mu_pos_samples[:,i] + noise_y * std_pos_samples[:,i]
y_samp_i_m = np.mean(y_samp_i)
# y_samp_i_m = np.quantile(y_samp_i, 0.5)
y_samp_i_q = np.quantile(y_samp_i, [0.025, 0.975])
y_stats[i,:] = np.concatenate(([y_samp_i_m],y_samp_i_q), axis=0)
###Output
_____no_output_____
###Markdown
Plot the resulting model95\% Bayesian credible interval of the posterior model.
###Code
# Plot training and test data
plt.plot(Xtrain, ytrain, 'bs', ms=6)
plt.plot(Xtest, ytest, 'gs', ms=3);
# Predictive mean
plt.plot(Xtest, f_stats[:,0], 'r--', lw=2)
# Shade in credible region
plt.fill_between(Xtest[:,0], y_stats[:,1], y_stats[:,2], alpha=0.1, color='blue')
plt.fill_between(Xtest[:,0], f_stats[:,1], f_stats[:,2], alpha=0.5)
plt.axis([-5, 5, -3, 3]);
plt.xlabel('x');
plt.ylabel('f, y');
plt.title('Samples from the GP posterior along with the training data');
###Output
_____no_output_____
###Markdown
Parameter learning: GPy with MLENow that we have considered how it works, we are allowed to cheat with the GPy library... GPy model
###Code
# Make a GP regression model
model = GPy.models.GPRegression(Xtrain,ytrain); # define model
model.optimize_restarts(num_restarts = 10); # optimize
display(model); # display resulting model
###Output
Optimization restart 1/10, f = -7.572121777524444
Optimization restart 2/10, f = -7.572121777527201
Optimization restart 3/10, f = -7.572121777534029
Optimization restart 4/10, f = -7.572121777534239
Optimization restart 5/10, f = -7.572121777533969
Optimization restart 6/10, f = -7.572121777534413
Optimization restart 7/10, f = -7.572121777532754
Optimization restart 8/10, f = -7.572121777527496
Optimization restart 9/10, f = -7.572121777534239
Optimization restart 10/10, f = -7.572121777533205
###Markdown
Plot the resulting modelSample from the posterior and 95\% Bayesian credible interval of the posterior model.
###Code
np.random.seed(1)
# Parameter estimates
sig_y, sig_f, l_f = np.sqrt(model.Gaussian_noise.variance[0]), np.sqrt(model.rbf.variance[0]), model.rbf.lengthscale[0]
print( 'Parameter estimates: ', np.array([sig_y, sig_f, l_f]) )
# Evaluate posterior
mu_pos, std_pos, f_pos = compute_pos1(Xtrain, ytrain, Xtest, sig_y, sig_f, l_f)
# plot posterior
plot_pos1(Xtrain, ytrain, Xtest, ytest, f_pos, mu_pos, std_pos)
###Output
Parameter estimates: [0.08986613 1.06315506 1.81767766]
###Markdown
Parameter learning: GPy with HMC inference The generative storyIn this section, we will use the following generative model in the HMC implementation;$$ p(\theta |\mathcal{D}) \propto p(\mathbf{y}|\mathbf{X},\boldsymbol\theta) p(\boldsymbol\theta), $$where $$ {\theta_i} = \text{Inv-Gamma}(2,2), $$and $\{\theta_i\}_{i=1}^3 = \{ \sigma^2, \sigma_f^2, l \}$. GPy model
###Code
# Make a GP regression model
model = GPy.models.GPRegression(Xtrain,ytrain)
# Give some general prior distributions for model parameters
model.kern.lengthscale.set_prior(GPy.priors.Gamma(2,1/2))
model.kern.variance.set_prior(GPy.priors.Gamma(2,1/2))
model.likelihood.variance.set_prior(GPy.priors.Gamma(2,1/2))
print(model)
###Output
reconstraining parameters GP_regression.rbf.lengthscale
reconstraining parameters GP_regression.rbf.variance
reconstraining parameters GP_regression.Gaussian_noise.variance
###Markdown
Sampling from the posterior distribution of model parametersThis shows how to sample from éne chain; in a real application, we would initialize multiple chains overdispersed in the plausible range of the variables and check for mixing.
###Code
hmc = GPy.inference.mcmc.HMC(model,stepsize=1e-1)
hmc_samp = hmc.sample(num_samples=1000)
###Output
_____no_output_____
###Markdown
Analyze samplesWe regard the first half of the samples as burn-in and plot the posterior samples (blue).
###Code
samples = hmc_samp[500:] # cut out the burn-in period
my_ind = [2,0,1] # my indexing
samples = samples[:,my_ind] # redefine samples with my indexing
plt.figure()
plt.hist(np.sqrt( samples[:,0] ));
plt.title('Gaussian noise standard deviation');
plt.figure()
plt.hist(np.sqrt( samples[:,1] ));
plt.title('RBF standard deviation');
plt.figure()
plt.hist( samples[:,2] );
plt.title('RBF lengthscale');
plt.plot(samples);
plt.title('Trace plots');
###Output
_____no_output_____
###Markdown
Posterior predictive distribution: Point estimateHere we pick óne parameter setting for the parameters. Plot the resulting modelSample from the posterior and 95\% Bayesian credible interval of the posterior model.
###Code
np.random.seed(1)
# Parameter estimates
par_est = (ss.mode(samples)[0][0]);
par_est[0:2] = np.sqrt(par_est[0:2]); print( 'Parameter estimates: ', par_est )
# Evaluate posterior
mu_pos, std_pos, f_pos = compute_pos1(Xtrain, ytrain, Xtest, par_est[0], par_est[1], par_est[2])
# plot posterior
plot_pos1(Xtrain, ytrain, Xtest, ytest, f_pos, mu_pos, std_pos)
###Output
Parameter estimates: [0.12649745 0.93352571 1.89423147]
###Markdown
Posterior predictive distribution: Full Bayesian inferenceHere we average over the posterior samples. Evaluate mean function and covariance for all posterior samples
###Code
samples[:,0:2] = np.sqrt( samples[:,0:2] )
n_samp = samples.shape[0]
m_points = Xtest.shape[0]
mu_pos_samples = np.zeros(( n_samp, m_points ))
std_pos_samples = np.zeros(( n_samp, m_points ))
for i in range(n_samp):
par_vec_i = samples[i,:]
amplitude = par_vec_i[1]; length_scale=par_vec_i[2]
K = se_kern(Xtrain, Xtrain, amplitude, length_scale)
K_s = se_kern(Xtrain, Xtest, amplitude, length_scale)
K_ss = se_kern(Xtest, Xtest, amplitude, length_scale)
# Apply the kernel function to our training points
L = np.linalg.cholesky(K + np.eye(nX_tr)*par_vec_i[0]**2)
# predictive mean
alp_m = np.linalg.solve(L.T,np.linalg.solve(L, ytrain))
mu_pos = np.dot(K_s.T, alp_m)
# predictive variance
vv_m = np.linalg.solve(L,K_s)
cov_pos = K_ss - vv_m.T.dot(vv_m)
std_pos = (np.diag(cov_pos)**(0.5)).reshape(-1,1)
mu_pos_samples[i,:] = mu_pos.T
std_pos_samples[i,:] = std_pos.T
###Output
_____no_output_____
###Markdown
Define sample statistics for the latent function (f) and the output (y)
###Code
f_stats = np.zeros((m_points,3))
y_stats = np.zeros((m_points,3))
noise_y = np.random.normal(np.zeros(n_samp))
for i in range(m_points):
f_samp_i = mu_pos_samples[:,i]
f_samp_i_m = np.mean(f_samp_i)
# f_samp_i_m = np.quantile(f_samp_i, 0.5)
f_samp_i_q = np.quantile(f_samp_i, [0.025, 0.975])
f_stats[i,:] = np.concatenate(([f_samp_i_m],f_samp_i_q), axis=0)
y_samp_i = mu_pos_samples[:,i] + noise_y * std_pos_samples[:,i]
y_samp_i_m = np.mean(y_samp_i)
# y_samp_i_m = np.quantile(y_samp_i, 0.5)
y_samp_i_q = np.quantile(y_samp_i, [0.025, 0.975])
y_stats[i,:] = np.concatenate(([y_samp_i_m],y_samp_i_q), axis=0)
###Output
_____no_output_____
###Markdown
Plot the resulting model95\% Bayesian credible interval of the posterior model.
###Code
# Plot training and test data
plt.plot(Xtrain, ytrain, 'bs', ms=6)
plt.plot(Xtest, ytest, 'gs', ms=3);
# Predictive mean
plt.plot(Xtest, f_stats[:,0], 'r--', lw=2)
# Shade in credible region
plt.fill_between(Xtest[:,0], y_stats[:,1], y_stats[:,2], alpha=0.1, color='blue')
plt.fill_between(Xtest[:,0], f_stats[:,1], f_stats[:,2], alpha=0.5)
plt.axis([-5, 5, -3, 3]);
plt.xlabel('x');
plt.ylabel('f, y');
plt.title('Samples from the GP posterior along with the training ');
###Output
_____no_output_____
###Markdown
Multi-output Gaussian Process RegressionSee Alvarez et al. (2012) for a reference on multi-output Gaussian processes. The modelIn this section, the framework presented in the foregoing section is extended to cover multi-output processes, thus the available data set for this case is $\mathcal{D}=\{ \mathbf{X}, \mathbf{Y} \} = \{ \mathbf{x}[n], \mathbf{y}[n] \}_{n=1}^N$, where both the inputs and outputs are vector-valued. Furthermore, in the further treatment, it is assumed that all inputs are applied in the regression for all outputs.In multi-output learning the output space is a vector space, thus leading to a vector-valued estimator $\mathbf{f}$, which is assumed to follow a GP:$$ \mathbf{f} \sim \mathcal{GP}(\mathbf{m}, \mathbf{K}), $$where $\mathbf{m}=\{m_d(\mathbf{x})\}_{d=1}^D$, i.e. the expected value functions of the outputs, and $\mathbf{K} = ( \mathbf{K}(\mathbf{x}, \mathbf{x}') )_{d,d'}$ is a matrix-valued function, such that the entries correspond to the covariances between the outputs $f_d(\mathbf{x})$ and $f_{d'}(\mathbf{x}')$. Under proper normalization of the data, the expected value functions can be assumed to be the zero vector without loss of generality, and the covariance function should then capture basic aspects of the process, such as stationarity and smoothness.The prior distribution over $\mathbf{f}$ takes the form:$$ \mathbf{f}(\mathbf{X}) \sim \mathsf{N}(\mathbf{m}(\mathbf{X}), \mathbf{K}(\mathbf{X}, \mathbf{X})), $$where $\mathbf{m}(\mathbf{X})$ is a vector that concatenates the expected value vectors of the outputs, and $\mathbf{K}(\mathbf{X}, \mathbf{X})$ is a block partitioned matrix defined as$$ \mathbf{K}(\mathbf{X}, \mathbf{X}) =\left[ \begin{array}{ccc} (\mathbf{K}(\mathbf{X},\mathbf{X}))_{1,1} & \cdots & (\mathbf{K}(\mathbf{X},\mathbf{X}))_{1,D} \\(\mathbf{K}(\mathbf{X},\mathbf{X}))_{2,1} & \cdots & (\mathbf{K}(\mathbf{X},\mathbf{X}))_{2,D} \\\vdots & \ddots & \vdots \\(\mathbf{K}(\mathbf{X},\mathbf{X}))_{D,1} & \cdots & (\mathbf{K}(\mathbf{X},\mathbf{X}))_{D,D} \\\end{array} \right]. $$If again a Gaussian likelihood model is assumed, i.e. $$ \mathbf{y} \sim \mathcal{N}(\mathbf{f}(\mathbf{x}), \Sigma) $$ with $\Sigma$ representing a diagonal matrix of elements $\{ \sigma_d \}_{d=1}^D$, the predictive distribution for a new data point $\mathbf{x}_*$ has a closed form solution:$$ p(\mathbf{f}(\mathbf{x}_*) | \mathcal{D}, \mathbf{x}_*, \boldsymbol\Theta) = \mathsf{N}(\mathbf{f}_*(\mathbf{x}_*), \mathbf{K}_*(\mathbf{x}_*, \mathbf{x}_*) ) $$where $\boldsymbol\Theta$ denotes the set of model parameters, and $\mathbf{f}(\mathbf{x}_*)$ and $\mathbf{K}_*(\mathbf{x}_*, \mathbf{x}_*)$ are defined as:$$ \mathbf{f}_*(\mathbf{x}_*) = \mathbf{K}_{\mathbf{x}_*}^T (\mathbf{K}(\mathbf{X},\mathbf{X})+\pmb{\Sigma})^{-1}\hat{\mathbf{y}}_c $$$$ \mathbf{K}_*(\mathbf{x}_*, \mathbf{x}_*) = \mathbf{K}(\mathbf{x}_*, \mathbf{x}_*) - \mathbf{K}_{\mathbf{x}_*}^T (\mathbf{K}(\mathbf{X},\mathbf{X})+\pmb{\Sigma})^{-1} \mathbf{K}_{\mathbf{x}_*}, $$with $\mathbf{y}_c$ being a vector of length $N \times D$ that concatenates the observed output vectors, $\pmb{\Sigma} = \Sigma \otimes \mathbf{I}_N$ is the Kronecker product between the noise covariance matrix and an identity matrix of size $N$, and $\mathbf{K}_{\mathbf{x}_*} = (\mathbf{K}(\mathbf{X}, \mathbf{x}_*))_{d,d'}$. In this tutorial, we will consider a separable matrix-valued kernel defined via the intrinsic coregionalization model (ICM):$$ (\mathbf{K}(\mathbf{x}, \mathbf{x}'))_{d,d'} = k_\mathbf{x}(\mathbf{x}, \mathbf{x}') k_\mathbf{y}(d,d'), $$where $k_\mathbf{x}$ and $k_\mathbf{y}$ encodes the covariances between the inputs and outputs, respectively. ***Alvarez, M. A., Rosasco, L., Lawrence, N. D. (2012). Kernels for vector-valued functions: A review.Founda-tions and Trends in Machine Learning, 4(3):195–266.*** Sample data
###Code
np.random.seed(42)
# Noise free/Noisy training data
nX_tr = 10
std_y = 1e-1 # if noise free set to 1e-4 for numerical stability; corr. var = 1e-16 addition along diagonal
XX = np.random.uniform(low=-4, high=1, size=(nX_tr,1)).reshape(-1,1)
YY = np.concatenate((np.sin(XX), np.sin(XX+np.pi/4)), axis=1) + std_y*np.random.normal(size=(nX_tr,1))
# Test data
nX_te = 50
XX_te = np.linspace(-5, 5, nX_te).reshape(-1,1)
YY_te = np.concatenate((np.sin(XX_te), np.sin(XX_te+np.pi/4)), axis=1) + std_y*np.random.normal(size=(nX_te,1))
# plot
plt.plot(XX, YY[:,0], 'bs', ms=6);
plt.plot(XX, YY[:,1], 'rs', ms=6);
plt.plot(XX_te, YY_te[:,0], 'bs', ms=3);
plt.plot(XX_te, YY_te[:,1], 'rs', ms=3);
plt.xlabel('x');
plt.ylabel('y');
plt.title('Training set');
###Output
_____no_output_____
###Markdown
Posterior model considering the processes jointlyThe posterior is formed as for single-output GPs by conditioning the prior over functions on the training data. Single-output quantities
###Code
amplitude = 1; length_scale=1.8
K = se_kern(XX, XX, amplitude, length_scale)
K_s = se_kern(XX, XX_te, amplitude, length_scale)
K_ss = se_kern(XX_te, XX_te, amplitude, length_scale)
K_y = np.array([[0.35, 0.25], [0.25, 0.5]]) # output kernel (true)
SIG_y = (std_y**2)*np.eye(2)
###Output
_____no_output_____
###Markdown
Multi-output quantitiesThe two processes in this example are observed at the same inputs, i.e. we study an isotopic multi-output case, as opposed to a situation where the outputs are observed at different inputs, which is referred to as heterotopic. Because we are studying the isotropic case, the multi-output quantities may conveniently be formed by Kronecker products of the single-output quantities, thus $k_x(x,x') k_y(d,d')$ is defined in terms of Kronecker products. Note that in the heterotrophic case $k_x(x,x') k_y(d,d')$ is defined in terms of tensor products, as $k_x(x,x')$ is defined for the specific combination of outputs, and not just repeated for all output combinations as for the isotropic case.
###Code
KK = np.kron(K_y,K) # compined Gram matrix
KK_s = np.kron(K_y,K_s)
KK_ss = np.kron(K_y,K_ss)
SIG_KK = np.kron( SIG_y , np.eye(nX_tr) )
Y_c = YY.ravel('F')[:, None]
###Output
_____no_output_____
###Markdown
Posterior predictive distribution95\% Bayesian credible interval of the posterior model.
###Code
# Apply the kernel function to our training points
L_KKy = np.linalg.cholesky(KK + SIG_KK)
invL_KKy = np.linalg.inv(L_KKy)
invKKy = invL_KKy.T.dot(invL_KKy) # invK = invL^T invL
# predictive mean
MU_c = KK_s.T.dot( invKKy ).dot( Y_c )
COV_c = KK_ss - (KK_s.T.dot( invKKy )).dot( KK_s )
STD_c = np.sqrt( np.diag(COV_c) )
MU = MU_c.reshape((2,nX_te)).T
STD = STD_c.reshape((2,nX_te)).T
plt.figure()
plt.plot(XX, YY[:,0], 'bs', ms=6);
plt.plot(XX, YY[:,1], 'rs', ms=6);
plt.plot(XX_te, YY_te[:,0], 'bs', ms=3);
plt.plot(XX_te, YY_te[:,1], 'rs', ms=3);
plt.fill_between(x=XX_te[:,0], y1=MU[:,0]-2*STD[:,0], y2=MU[:,0]+2*STD[:,0], alpha=0.1, color='blue');
plt.plot(XX_te, MU[:,0], 'b--', lw=2)
plt.fill_between(x=XX_te[:,0], y1=MU[:,1]-2*STD[:,1], y2=MU[:,1]+2*STD[:,1], alpha=0.1, color='red');
plt.plot(XX_te, MU[:,1], 'r--', lw=2)
plt.axis([-5, 5, -2.5, 2.5]);
plt.xlabel('x')
plt.ylabel('f,y')
plt.title('GP posterior model along with training data');
# plt.show()
###Output
_____no_output_____
###Markdown
As we have considered a zero mean function for the processes, the predictions will simply approach zero, as the distance to the training examples gets larger, see e.g. x=5, thus this model should only be use as an interpolator or for extrapolation near the training points, as is also apparent from the plot. Posterior model considering the processes separately95\% Bayesian credible interval of the posterior model.
###Code
# Apply the kernel function to our training points
Ky = K + (sig_y**2)*np.eye(nX_tr)
Ly = np.linalg.cholesky(Ky)
# inverse of covariance
invLy = np.linalg.inv(Ly)
invKy = invLy.T.dot(invLy) # invK = invL^T invL
# alpha
alp_y1 = invKy.dot(YY[:,0])
alp_y2 = invKy.dot(YY[:,1])
# predictive mean
mu_pos1 = np.dot(K_s.T, alp_y1)
mu_pos2 = np.dot(K_s.T, alp_y2)
# predictive variance
vv_m = np.linalg.solve(Ly,K_s)
cov_pos = K_ss - vv_m.T.dot(vv_m)
std_pos = (np.diag(cov_pos)**(0.5)).reshape(-1,1) # may need abs as variance is zero at training points (no error), which may be
plt.figure()
plt.plot(XX, YY[:,0], 'bs', ms=6);
plt.plot(XX, YY[:,1], 'rs', ms=6);
plt.plot(XX_te, YY_te[:,0], 'bs', ms=3);
plt.plot(XX_te, YY_te[:,1], 'rs', ms=3);
plt.fill_between(x=XX_te[:,0], y1=mu_pos1-2*std_pos[:,0], y2=mu_pos1+2*std_pos[:,0], alpha=0.1, color='blue');
plt.plot(XX_te, mu_pos1, 'b--', lw=2)
plt.fill_between(x=XX_te[:,0], y1=mu_pos2-2*std_pos[:,0], y2=mu_pos2+2*std_pos[:,0], alpha=0.1, color='red');
plt.plot(XX_te, mu_pos2, 'r--', lw=2)
plt.axis([-5, 5, -2.5, 2.5]);
plt.xlabel('x')
plt.ylabel('f,y')
plt.title('GP posterior model along with training data');
# # plt.show()
###Output
_____no_output_____
###Markdown
Note that the uncertainty bounds are wider when considering the two processes separately! Thus, when the two processes are considered jointly, they borrow statistical power from each other through the output kernel $k_y(d,d')$, which expresses the output correlation. Parameter learning: GPy with MLELearning of multi-output GPs follow the same approaches as explained for single-output GPs above, so we will simply show how this can be done using GPy to minimize repetition. Intrinsic Coregionalization Model (ICM) Re-structure the data into the format GPy expects
###Code
Xgpy = np.vstack([
np.vstack([XX[:,0], np.ones_like(XX[:,0])*0]).T,
np.vstack([XX[:,0], np.ones_like(XX[:,0])*1]).T
])
ygpy = np.hstack([
YY[:,0].T,
YY[:,1].T
])
ygpy = ygpy.reshape((len(ygpy),1))
###Output
_____no_output_____
###Markdown
Define ICM kernel
###Code
# icm = GPy.kern.RBF(input_dim=1)**GPy.kern.Coregionalize(input_dim=1,output_dim=2, rank=2)
icm = GPy.util.multioutput.ICM(input_dim=1,num_outputs=2,kernel=GPy.kern.RBF(1),W_rank=2)
display(icm)
###Output
_____no_output_____
###Markdown
Fit model
###Code
model = GPy.models.GPRegression(Xgpy, ygpy, icm)
model.optimize_restarts(num_restarts = 10);
out_sy=np.sqrt(model.Gaussian_noise.variance[0]); print(out_sy)
rbf_sf=np.sqrt(model.ICM.rbf.variance[0]); print(rbf_sf)
rbf_lf=(model.ICM.rbf.lengthscale[0]); print(rbf_lf)
coteg_B=model.ICM.B.B; print(coteg_B)
###Output
0.07917120780962851
0.7651946499645894
1.7337299086051674
[[1.51577785 0.97167378]
[0.97167378 1.22905807]]
###Markdown
Plot model fit
###Code
amplitude = rbf_sf; length_scale=rbf_lf
K = se_kern(XX, XX, amplitude, length_scale)
K_s = se_kern(XX_te, XX, amplitude, length_scale)
K_ss = se_kern(XX_te, XX_te, amplitude, length_scale)
K_y = coteg_B
SIG_y = (out_sy**2)*np.eye(2)
KK = np.kron(K_y,K) # compined Gram matrix
KK_s = np.kron(K_y,K_s)
KK_ss = np.kron(K_y,K_ss)
SIG_KK = np.kron( SIG_y , np.eye(nX_tr) )
Y_c = YY.ravel('F')[:, None]
# Apply the kernel function to our training points
L_KKy = np.linalg.cholesky(KK + SIG_KK)
invL_KKy = np.linalg.inv(L_KKy)
invKKy = invL_KKy.T.dot(invL_KKy) # invK = invL^T invL
# predictive mean
MU_c = KK_s.dot( invKKy ).dot( Y_c )
COV_c = KK_ss - (KK_s.dot( invKKy )).dot( KK_s.T )
STD_c = np.sqrt( np.diag(COV_c) )
MU = MU_c.reshape((2,nX_te)).T
STD = STD_c.reshape((2,nX_te)).T
plt.figure()
plt.plot(XX, YY[:,0], 'bs', ms=6);
plt.plot(XX, YY[:,1], 'rs', ms=6);
plt.plot(XX_te, YY_te[:,0], 'bs', ms=3);
plt.plot(XX_te, YY_te[:,1], 'rs', ms=3);
plt.fill_between(x=XX_te[:,0], y1=MU[:,0]-2*STD[:,0], y2=MU[:,0]+2*STD[:,0], alpha=0.1, color='blue');
plt.plot(XX_te, MU[:,0], 'b--', lw=2)
plt.fill_between(x=XX_te[:,0], y1=MU[:,1]-2*STD[:,1], y2=MU[:,1]+2*STD[:,1], alpha=0.1, color='red');
plt.plot(XX_te, MU[:,1], 'r--', lw=2)
plt.axis([-5, 5, -2.5, 2.5]);
plt.xlabel('x')
plt.ylabel('f,y')
plt.title('GP posterior model along with training data');
# # plt.show()
# Build-in GPy function
fig, ax = plt.subplots()
for i in range(2):
model.plot_latent(fignum=1,plot_limits=np.array([-5,5]),fixed_inputs=[(1, i)],ax=ax,legend=False)
plt.xlabel('x')
plt.ylabel('f')
plt.ylim([-2.5, 2.5]);
# plt.grid();
###Output
_____no_output_____
###Markdown
Independent models Define and fit models
###Code
model1 = GPy.models.GPRegression(XX,YY[:,0].reshape(-1,1))
model2 = GPy.models.GPRegression(XX,YY[:,1].reshape(-1,1))
model1.optimize_restarts(num_restarts = 5);
model2.optimize_restarts(num_restarts = 5);
###Output
Optimization restart 1/5, f = -0.48918739810105905
Optimization restart 2/5, f = -0.4891873981105217
Optimization restart 3/5, f = -0.489187398114713
Optimization restart 4/5, f = -0.4891873981144812
Optimization restart 5/5, f = -0.4891873981142991
Optimization restart 1/5, f = -1.0500999733516387
Optimization restart 2/5, f = -1.0500999733522232
Optimization restart 3/5, f = -1.0500999733522551
Optimization restart 4/5, f = -1.0500999733537038
Optimization restart 5/5, f = -1.0500999733529373
###Markdown
Plot model fits
###Code
# Model 1
sig_y, sig_f, l_f = np.sqrt(model1.Gaussian_noise.variance[0]), np.sqrt(model1.rbf.variance[0]), model1.rbf.lengthscale[0]
K_s = se_kern(XX, XX_te, sig_f, l_f)
K_ss = se_kern(XX_te, XX_te, sig_f, l_f)
# Apply the kernel function to our training points
Ky = Ky_kern(XX, XX, sig_y, sig_f, l_f)
Ly = np.linalg.cholesky(Ky)
# inverse of covariance
invLy = np.linalg.inv(Ly)
invKy = invLy.T.dot(invLy) # invK = invL^T invL
# alpha
alp_y = invKy.dot(YY[:,0].reshape(-1,1))
# predictive mean
mu_pos1 = np.dot(K_s.T, alp_y)
# predictive variance
vv_m = np.linalg.solve(Ly,K_s)
cov_pos = K_ss - vv_m.T.dot(vv_m)
std_pos1 = (np.diag(cov_pos)**(0.5)).reshape(-1,1) # may need abs as variance is zero at training points (no error), which may be encoded as -0 (no allowed)
# Model 2
sig_y, sig_f, l_f = np.sqrt(model2.Gaussian_noise.variance[0]), np.sqrt(model2.rbf.variance[0]), model2.rbf.lengthscale[0]
K_s = se_kern(XX, XX_te, sig_f, l_f)
K_ss = se_kern(XX_te, XX_te, sig_f, l_f)
# Apply the kernel function to our training points
Ky = Ky_kern(XX, XX, sig_y, sig_f, l_f)
Ly = np.linalg.cholesky(Ky)
# inverse of covariance
invLy = np.linalg.inv(Ly)
invKy = invLy.T.dot(invLy) # invK = invL^T invL
# alpha
alp_y = invKy.dot(YY[:,1].reshape(-1,1))
# predictive mean
mu_pos2 = np.dot(K_s.T, alp_y)
# predictive variance
vv_m = np.linalg.solve(Ly,K_s)
cov_pos = K_ss - vv_m.T.dot(vv_m)
std_pos2 = (np.diag(cov_pos)**(0.5)).reshape(-1,1) # may need abs as variance is zero at training points (no error), which may be encoded as -0 (no allowed)
# Plot
plt.figure()
plt.plot(XX, YY[:,0], 'bs', ms=6);
plt.plot(XX, YY[:,1], 'rs', ms=6);
plt.plot(XX_te, YY_te[:,0], 'bs', ms=3);
plt.plot(XX_te, YY_te[:,1], 'rs', ms=3);
plt.fill_between(x=XX_te[:,0], y1=mu_pos1[:,0]-2*std_pos1[:,0], y2=mu_pos1[:,0]+2*std_pos1[:,0], alpha=0.1, color='blue');
plt.plot(XX_te, mu_pos1, 'b--', lw=2)
plt.fill_between(x=XX_te[:,0], y1=mu_pos2[:,0]-2*std_pos2[:,0], y2=mu_pos2[:,0]+2*std_pos2[:,0], alpha=0.1, color='red');
plt.plot(XX_te, mu_pos2, 'r--', lw=2)
plt.axis([-5, 5, -2.5, 2.5]);
plt.xlabel('x')
plt.ylabel('f,y')
plt.title('Samples from the GP posterior along with the training ');
# plt.show()
# Build-in GPy function
fig, ax = plt.subplots()
model1.plot_latent(fignum=1,plot_limits=np.array([-5,5]),ax=ax, legend=False)
model2.plot_latent(fignum=1,plot_limits=np.array([-5,5]),ax=ax, legend=False)
plt.xlabel('x')
plt.ylabel('f')
plt.ylim([-2.5, 2.5]);
# plt.grid();
###Output
_____no_output_____ |
intermediate-lessons/interdisciplinary-communication/ic-1.ipynb | ###Markdown
Interdisciplinary CommunicationThis Intermediate lesson on Interdisciplinary Communication introduces ...Lesson Developers:
###Code
# This code cell starts the necessary setup for Hour of CI lesson notebooks.
# First, it enables users to hide and unhide code by producing a 'Toggle raw code' button below.
# Second, it imports the hourofci package, which is necessary for lessons and interactive Jupyter Widgets.
# Third, it helps hide/control other aspects of Jupyter Notebooks to improve the user experience
# This is an initialization cell
# It is not displayed because the Slide Type is 'Skip'
from IPython.display import HTML, IFrame, Javascript, display
from ipywidgets import interactive
import ipywidgets as widgets
from ipywidgets import Layout
import getpass # This library allows us to get the username (User agent string)
# import package for hourofci project
import sys
sys.path.append('../../supplementary') # relative path (may change depending on the location of the lesson notebook)
import hourofci
# Retreive the user agent string, it will be passed to the hourofci submit button
agent_js = """
IPython.notebook.kernel.execute("user_agent = " + "'" + navigator.userAgent + "'");
"""
Javascript(agent_js)
# load javascript to initialize/hide cells, get user agent string, and hide output indicator
# hide code by introducing a toggle button "Toggle raw code"
HTML('''
<script type="text/javascript" src=\"../../supplementary/js/custom.js\"></script>
<style>
.output_prompt{opacity:0;}
</style>
<input id="toggle_code" type="button" value="Toggle raw code">
''')
###Output
_____no_output_____ |
AH_210724_1.ipynb | ###Markdown
###Code
###Output
_____no_output_____ |
Hindi.ipynb | ###Markdown
Task 1: Word Embeddings (10 points)This notebook will guide you through all steps necessary to train a word2vec model (Detailed description in the PDF). ImportsThe following are the packages used
###Code
# Imports
import torch
torch.manual_seed(10)
from torch.autograd import Variable
import pandas as pd
import numpy as np
import sklearn as sk
import re
import itertools
import warnings
warnings.filterwarnings("ignore")
import nltk
import torch.nn as nn
import torch.optim as optim
import torch.nn.functional as F
###Output
_____no_output_____
###Markdown
1.1 Get the data (0.5 points)Load it into a data structure of your choice. Then, split off a small part of the corpus as a development set (~100 data points).The file hindi_hatespeech.tsv is uploaded into a variable "data". The data from columns - "text" and "task_1" is extracted and saved into X_TRAIN and Y_LABEL respectively.
###Code
#TODO: implement!
from google.colab import files
uploaded = files.upload()
#load data
data = pd.read_csv("hindi_hatespeech.tsv",sep='\t')
#split off a small part of the corpus as a development set (~100 data points)
from sklearn.model_selection import train_test_split
train_dev,test_dev = train_test_split(data,train_size=0.025,random_state=123) #random split off the dataset
y_label_dev = train_dev['task_1'].values #extract labels of development set
x_train_dev = train_dev['text'].values #extract sentences of development set
print(len(y_label_dev))
from sklearn.model_selection import train_test_split
train_dev1,test_dev = train_test_split(data,train_size=0.5,random_state=123)
#more data trained
X_TRAIN = train_dev1['text'].values # full dataset for sentence
Y_LABEL = train_dev1['task_1'].values # full dataset for labels
#extract label of dataset
def data_to_label(label):
y_number = []
for i in range(len(label)):
if label[i]=='HOF':
y_number.append(1)
elif label[i]=='NOT':
y_number.append(0)
return y_number
#assign numbers of labels of development set
# train the full data set
y_train = data_to_label(Y_LABEL)
result = pd.value_counts(y_train)
print(result)
###Output
1 1223
0 1109
dtype: int64
###Markdown
1.2 Data preparation (0.5 + 0.5 points)* Prepare the data by removing everything that does not contain information. User names (starting with '@') and punctuation symbols clearly do not convey information, but we also want to get rid of so-called [stopwords](https://en.wikipedia.org/wiki/Stop_word), i. e. words that have little to no semantic content (and, but, yes, the...). Hindi stopwords can be found [here](https://github.com/stopwords-iso/stopwords-hi/blob/master/stopwords-hi.txt) Then, standardize the spelling by lowercasing all words.Do this for the development section of the corpus for now.* What about hashtags (starting with '') and emojis? Should they be removed too? Justify your answer in the report, and explain how you accounted for this in your implementation.
###Code
#TODO: implement!
#clean the development data set
uploaded = files.upload()
stopwords = pd.read_csv('stopwords-hi.txt',header=None)
stop_words=stopwords[0].tolist()
punc=r'''!()-[]{};:'"\,<>./?@#$%^&*_“~'''
new_list=[]
for i in range(0,len(x_train_dev)):
# Punctuations removal
new=' '.join(word for word in x_train_dev[i].split() if word[0] not in punc)
new = ' '.join(re.sub("(\w+:\/\/\S+)", " ", new).split())
new = ' '.join(re.sub(r"\b\d+\b", " ", new).split())
new = ' '.join(re.sub("[\.\,\!\?\:\;\-\=\#\%\…\\u200d\।।]", " ", new).split())
new = ' '.join(re.sub("[\U0001F600-\U0001F64F]"," ",new).split()) # emotions
new = ' '.join(re.sub("[\U0001F300-\U0001F5FF]"," ",new).split()) # symbols & pictographs
new = ' '.join(re.sub("[\U0001F680-\U0001F6FF]"," ",new).split()) # transport & map symbols
new = ' '.join(re.sub("[\U0001F1E0-\U0001F1FF]"," ",new).split()) # flags (iOS)
new = ' '.join(re.sub("[\U00002702-\U000027B0]"," ",new).split())
new = ' '.join(re.sub("[\U000024C2-\U0001F251]"," ",new).split())
new = ' '.join(re.sub("[\U00001F92C]"," ",new).split())
# Converting into lowercase
new= new.lower()
# Removing stop words
new=' '.join(word for word in new.split() if word not in stop_words)
# Appending to the text list
new_list.append(new)
#final_data_dev=pd.Series(new_list,dtype="string")
print(len(new_list))
uploaded = files.upload()
stopwords = pd.read_csv('stopwords-hi.txt',header=None)
#clean the full data set
def clean_the_data(data):
new_list=[]
punc=r'''!()-[]{};:'"\,<>./?@#$%^&*_“~'''
stop_words=stopwords[0].tolist()
for i in range(0,len(data)):
# Punctuations removal
new=' '.join(word for word in data[i].split() if word[0] not in punc)
new = ' '.join(re.sub("(\w+:\/\/\S+)", " ", new).split())
new = ' '.join(re.sub(r"\b\d+\b", " ", new).split())
new = ' '.join(re.sub("[\.\,\!\?\:\;\-\=\#\%\…\\u200d\।।]", " ", new).split())
# Converting into lowercase
new= new.lower()
# Removing stop words
new=' '.join(word for word in new.split() if word not in stop_words)
# Appending to the text list
new_list.append(new)
return new_list
new_list=clean_the_data(X_TRAIN)
print(new_list[0])
nltk.download('punkt')
# Tokenizes each sentence by implementing the nltk tool
new_list_new = [nltk.tokenize.word_tokenize(x) for x in new_list]
###Output
_____no_output_____
###Markdown
1.3 Build the vocabulary (0.5 + 0.5 points)The input to the first layer of word2vec is an one-hot encoding of the current word. The output of the model is then compared to a numeric class label of the words within the size of the skip-gram window. Now* Compile a list of all words in the development section of your corpus and save it in a variable ```V```.
###Code
#TODO: implement!
V = {}
i=0
for s in range(len(new_list_new)):
n=new_list_new[s]
for y in range(len(n)):
w=new_list_new[s][y]
if w not in V:
V[w] = i
i+=1
y+=1
s+=1
print(len(V))
###Output
11138
###Markdown
* Then, write a function ```word_to_one_hot``` that returns a one-hot encoding of an arbitrary word in the vocabulary. The size of the one-hot encoding should be ```len(v)```.
###Code
#TODO: implement!
# translate words to integer numbers
def word_to_one_hot(word):
words = V.keys()
str_to_int = dict((c, i) for i, c in enumerate(words))
integer_encoded = [str_to_int[string] for string in [word]]
# one hot encode
onehot_encoded = []
for value in integer_encoded:
letter = [0 for _ in range(len(V))]
letter[value] = 1
onehot_encoded.append(letter)
#onehot_encoded.long()
return onehot_encoded
pass
#a=word_to_one_hot(new_list_new[1][5])
#print(a)
#修改一下变量名称
###Output
_____no_output_____
###Markdown
1.4 Subsampling (0.5 points)The probability to keep a word in a context is given by:$P_{keep}(w_i) = \Big(\sqrt{\frac{z(w_i)}{0.001}}+1\Big) \cdot \frac{0.001}{z(w_i)}$Where $z(w_i)$ is the relative frequency of the word $w_i$ in the corpus. Now,* Calculate word frequencies* Define a function ```sampling_prob``` that takes a word (string) as input and returns the probabiliy to **keep** the word in a context.
###Code
#TODO: implement!
Words = {}
i=0
for s in range(len(new_list_new)):
n=new_list_new[s]
for y in range(len(n)):
w=new_list_new[s][y]
Words[w] = i
i+=1
y+=1
s+=1
W2=list(Words)
def sampling_prob(word):
frac = W2.count(word)/len(W2)
prob = (np.sqrt(frac/0.000001) + 1) * (0.000001/frac)
return prob
pass
###Output
_____no_output_____
###Markdown
1.5 Skip-Grams (1 point)Now that you have the vocabulary and one-hot encodings at hand, you can start to do the actual work. The skip gram model requires training data of the shape ```(current_word, context)```, with ```context``` being the words before and/or after ```current_word``` within ```window_size```. * Have closer look on the original paper. If you feel to understand how skip-gram works, implement a function ```get_target_context``` that takes a sentence as input and [yield](https://docs.python.org/3.9/reference/simple_stmts.htmlthe-yield-statement)s a ```(current_word, context)```.* Use your ```sampling_prob``` function to drop words from contexts as you sample them.
###Code
#TODO: implement!
def get_target_context(sentence):
word_lists=[]
for i in range(len(sentence)):
w=sentence[i]
p_sample = sampling_prob(w)
threshold = np.random.random()
#print(threshold)
if p_sample > threshold:
# the word is kept
for n in range(2):
# look back
if (i-n-1)>=0:
word_lists.append([w] + [sentence[i-n-1]])
# look forward
if (i+n+1)<len(sentence):
word_lists.append([w]+[sentence[i+n+1]])
else:
# the word is dropped
i+=1
return word_lists
pass
###Output
_____no_output_____
###Markdown
1.6 Hyperparameters (0.5 points)According to the word2vec paper, what would be a good choice for the following hyperparameters? * Embedding dimension* Window sizeInitialize them in a dictionary or as independent variables in the code block below.
###Code
# Set hyperparameters
window_size = 2
embedding_size = 64
# More hyperparameters
learning_rate = 0.05
epochs = 10
###Output
_____no_output_____
###Markdown
1.7 Pytorch Module (0.5 + 0.5 + 0.5 points)Pytorch provides a wrapper for your fancy and super-complex models: [torch.nn.Module](https://pytorch.org/docs/stable/generated/torch.nn.Module.html). The code block below contains a skeleton for such a wrapper. Now,* Initialize the two weight matrices of word2vec as fields of the class.* Override the ```forward``` method of this class. It should take a one-hot encoding as input, perform the matrix multiplications, and finally apply a log softmax on the output layer.* Initialize the model and save its weights in a variable. The Pytorch documentation will tell you how to do that.
###Code
vocabulary_size=len(V)
class Word2Vec(nn.Module):
def __init__(self, embed_size, vocab_size):
super(Word2Vec, self).__init__()
self.input = nn.Embedding(vocab_size, embedding_size)
self.output = nn.Linear(embedding_size, vocab_size,bias=False)
def forward(self, one_hot):
#one_hot = torch.tensor(one_hot)
emb = self.input(one_hot)
hidden = self.output(emb)
out = F.log_softmax(hidden)
return out
# Initialize model
net = Word2Vec(embed_size=embedding_size, vocab_size=vocabulary_size)
device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
net = net.to(device)
#W1 = net.input.weight
#W2 = net.output.weight
#print(torch.cuda.is_available())
#M = word_to_one_hot('रन')
###Output
_____no_output_____
###Markdown
1.8 Loss function and optimizer (0.5 points)Initialize variables with [optimizer](https://pytorch.org/docs/stable/optim.htmlmodule-torch.optim) and loss function. You can take what is used in the word2vec paper, but you can use alternative optimizers/loss functions if you explain your choice in the report.
###Code
# Define optimizer and loss
optimizer = torch.optim.SGD(net.parameters(), lr=learning_rate)
criterion = nn.CrossEntropyLoss()
###Output
_____no_output_____
###Markdown
1.9 Training the model (3 points)As everything is prepared, implement a training loop that performs several passes of the data set through the model. You are free to do this as you please, but your code should:* Load the weights saved in 1.6 at the start of every execution of the code block* Print the accumulated loss at least after every epoch (the accumulate loss should be reset after every epoch)* Define a criterion for the training procedure to terminate if a certain loss value is reached. You can find the threshold by observing the loss for the development set.You can play around with the number of epochs and the learning rate.
###Code
# load initial weights
window_size = 2
embedding_size = 64
i=0
losses = [torch.tensor(1., device=device)]
#losses.append(1)
losses_mean=np.mean([tensor.cpu() for tensor in losses])
def train():
print("Training started")
train()
for epo in range(epochs):
while losses_mean> 0.001:
losses_mean=np.mean([tensor.cpu() for tensor in losses])
#mean = torch.mean(torch.stack(losses))
#mean = mean.to(device)
print("Loss: ", losses_mean)
net.train()
for i in range(len(new_list_new)):
# Define train procedure
# step1:Skip-Grams
sentence = new_list_new[i]
idx_pairs = get_target_context(sentence)
for target, context in idx_pairs:
# step2:target one-hot encoding
X = word_to_one_hot(target)
X = torch.tensor(X)
x1 = X[0]
x1 = x1.to(device)
#print(x1)
# step3:Word2Vec
y =net.forward(x1)
Y = word_to_one_hot(context)
Y = Y[0]
y_ture = torch.tensor(Y)
y_ture = y_ture.to(device)
# step4:loss
loss = criterion(y,y_ture)
#print(loss)
losses.append(loss.data)
losses.pop(0)
optimizer.zero_grad()
loss.backward()
optimizer.step()
# step5:Backprop to update model parameters
print("Training finished")
torch.save(net.state_dict(),'netweight1.pt')
net.load_state_dict(torch.load('netweight1.pt'))
net.eval()
W1=net.input.weight
print(net.input.weight)
###Output
Training started
Loss: 1.0
Loss: 0.0021761528
Loss: 0.0014511059
Loss: 0.0010456638
Loss: 0.0009634892
###Markdown
1.10 Train on the full dataset (0.5 points)Now, go back to 1.1 and remove the restriction on the number of sentences in your corpus. Then, reexecute code blocks 1.2, 1.3 and 1.6 (or those relevant if you created additional ones). * Then, retrain your model on the complete dataset.* Now, the input weights of the model contain the desired word embeddings! Save them together with the corresponding vocabulary items (Pytorch provides a nice [functionality](https://pytorch.org/tutorials/beginner/saving_loading_models.html) for this).
###Code
#torch.save(net.state_dict(), '/content/drive/MyDrive/Colab Notebooks')
torch.save(net.state_dict(),'netweight1.pt')
net.load_state_dict(torch.load('netweight1.pt'))
net.eval()
W1=net.input.weight
print(net.input.weight)
###Output
Parameter containing:
tensor([[-0.8173, -0.5556, -0.8267, ..., 0.1497, -0.2460, -1.4636],
[ 0.5876, -1.1603, 1.0045, ..., 0.5878, 0.5066, -1.2699],
[-0.1746, 1.1172, 0.8670, ..., -0.2765, -2.4868, -1.0496],
...,
[ 0.4476, 0.4974, 0.5029, ..., -1.5684, -0.4717, 1.1181],
[ 1.5851, 0.9026, 2.1100, ..., -0.5175, -0.2344, -0.1135],
[ 0.1542, -0.4143, 0.0274, ..., -0.1400, -0.1323, 0.0228]],
device='cuda:0', requires_grad=True)
###Markdown
Task 2.1 Binary neural sentiment classifier
###Code
Weight3=[]
for i in range(len(V)-1):
weight3=[]
w=W1[i]
for y in range(embedding_size):
wei=w[y].item()
weight3.append(wei)
Weight3.append(weight3)
V2 = dict(zip(V, Weight3))
print(len(V2))
###Output
11137
###Markdown
The unique words are 11,137 in the vocabulary.
###Code
sentence_padding =[]
pad_idx = 0
padding_standard = max(new_list_new, key=len,default='')
#padding the sentence to the same length
for i in range(len(new_list_new)):
temp_sentence = list()
temp = new_list_new[i]
while len(temp) < len(padding_standard):
temp.insert(len(temp), pad_idx)
sentence_padding.append(temp)
#make sentences to the same size matrix using word embedding expression
sentence_train=[]
for i in range(len(sentence_padding)):
temp_sentence = list()
temp = new_list_new[i]
for word in temp:
if word in V2.keys():
temp_sentence.append(V2[word])
else:
temp_sentence.append(np.zeros(embedding_size))# 可能需要修改
sentence_train.append(temp_sentence)
print(np.shape(sentence_train))
sentence_train3=torch.tensor(sentence_train)
from google.colab import drive
drive.mount('/content/drive')
import sys
sys.path.append('/content/drive/MyDrive/Colab Notebooks')
from modelinput import CNN
EMBEDDING_DIM = embedding_size
N_FILTERS = 100
FILTER_SIZES = [2,3,4]
OUTPUT_DIM = 1
DROPOUT = 0.5
model = CNN(EMBEDDING_DIM, N_FILTERS, FILTER_SIZES, OUTPUT_DIM, DROPOUT)
optimizer1 = optim.Adam(model.parameters())
criterion1 = nn.BCEWithLogitsLoss()
model = model.to(device)
criterion1 = criterion1.to(device)
from modelinput import binary_accuracy
N_EPOCHS = 50
sentence_train3=sentence_train3.to(device,dtype=torch.float)
Y_train = torch.tensor(y_train).to(device)
for epoch in range(N_EPOCHS):
epoch_loss = 0
epoch_acc = 0
model.train()
optimizer1.zero_grad()
predictions = model.forward(sentence_train3).squeeze(1)
loss1 = criterion1(predictions, Y_train.float())
#print(np.shape(predictions))
acc = binary_accuracy(predictions, Y_train)
loss1.backward()
optimizer1.step()
epoch_loss += loss1.item()
epoch_acc += acc.item()
print(f'\tTrain Loss: {loss1:.3f} | Train Acc: {acc*100:.2f}%')
def evaluate(model):
epoch_loss = 0
epoch_acc = 0
model.eval()
predictions = model(sentence_train3).squeeze(1)
loss = criterion1(predictions, Y_train.float())
acc = binary_accuracy(predictions, Y_train)
epoch_loss += loss.item()
epoch_acc += acc.item()
return epoch_loss, epoch_acc
test_loss, test_acc = evaluate(model)
print(f'Test Loss: {test_loss:.3f} | Test Acc: {test_acc*100:.2f}%')
torch.save(model.state_dict(),'CNNmodelweight.pt')
###Output
_____no_output_____
###Markdown
Task3 Transformer Implement
###Code
import torch.nn as nn
import copy
def clones(module, N):
"Produce N identical layers."
return nn.ModuleList([copy.deepcopy(module) for _ in range(N)])
import math,copy
#doing the position encoding first
def positionalencoding1d(d_model, length):
if d_model % 2 != 0:
raise ValueError("Cannot use sin/cos positional encoding with "
"odd dim (got dim={:d})".format(d_model))
pe = torch.zeros(length, d_model)
position = torch.arange(0, length).unsqueeze(1)
div_term = torch.exp((torch.arange(0, d_model, 2, dtype=torch.float) *
-(math.log(10000.0) / d_model)))
pe[:, 0::2] = torch.sin(position.float() * div_term)
pe[:, 1::2] = torch.cos(position.float() * div_term)
return pe
posit = positionalencoding1d(64,61) # the shape of one padding sentence
posit = torch.tensor(posit,device=device)
AttInput=torch.empty(np.shape(sentence_train3))
for i in range(len(sentence_train3)):
tar =sentence_train3[i]
AttInput[i]= tar+posit
Input = AttInput[0:100,]
SRC_VOCAB=1
N_CLASS=1
D_MODEL=embedding_size
D_FF=1024
N = 6
H=8
DROP_OUT=0.1
import modelinput
model2 = modelinput.make_model(SRC_VOCAB,N,D_MODEL,D_FF,H,DROP_OUT, N_CLASS)
model2 = model2.to(device)
lr=0.005
criterion2 = nn.CrossEntropyLoss()
optimizer2 = torch.optim.Adam(model2.parameters(),lr)
N_EPOCHS = 10
torch.cuda.empty_cache()
torch.cuda.empty_cache()
for epoch in range(N_EPOCHS):
epoch_loss2 = 0
epoch_acc2 = 0
optimizer2.zero_grad()
x = AttInput.to(device)
y = torch.tensor(y_train, dtype=torch.long, device=device)
#y = y.unsqueeze(1)
output = model2(x, None)
loss2 = criterion2(output,y)
loss2.backward()
optimizer2.step()
epoch_loss2 += loss2.item()
print(f'\tTrain Loss: {loss2:.3f}')
###Output
_____no_output_____ |
site/public/courses/DS-1.1/Notebooks/ConditionalProbability.ipynb | ###Markdown
Probability refresherSuggested readings before class:[Math is fun:Probability](https://www.mathsisfun.com/data/probability.html)Probability is all about the **chances of an event occuring** or how likely an event is to occur, in a set of events.If you really think about it, you've probably been thinking about probability all of your life, such as if you've ever wonderered about > - The chances of it raining today> - The chances of winning the lottery> - The chances of getting hired at GoogleTo really make sense of **the chances** of an event occuring, we need to look at a bit of math through **probability**.In math probability is modeled by the expression:$P(A)= \frac{Count of A }{sample Space}$ $ P $ is the probability of the event $ A $ occuring in a set of observed events $ sample space $$ count of A $ - is the number of times an certain detail was present in the whole set$ sampleSpace $ - total number of observed eventsTo better understand how works, the closer this number is to 0, the less likely it is to occur, with a value 0 meaning it didnt happen at all. The closer to 1 being an indicator that it, the event is more likely to happen, with a value of 1 being that in every single case this happened.You'll often see this represented in data sets in a number of formats. Here are some examples:| Hired||------|| false|| true || true || false|| Won Lottery || ----|| yes || no || no || no || Survived || ----|| 0 || 1 || 0 || 1 |Lets look at a short example by actually examining some hiring numbers at Google[Click here to read an article about the hiring stats at Google](https://qz.com/285001/heres-why-you-only-have-a-0-2-chance-of-getting-hired-at-google/)> __Google gets around 3 million applications a year now, according to HR head Laszlo Bock, and hires 7,000 .... making it far more selective than institutions like Harvard, Yale, and Stanford.__So we have 3 million **observed events**,(in this case the event is submitting an application).And in 7000 of those 3 million them a hiring occuredLets model that with some code!
###Code
# total number of applicants to Google
num_of_applicants = 3000000
# here we have the number of people that actually got hired out of that group
total_hires = 7000
def probability(event_count, sample_space):
p = event_count/sample_space
return p
prob = probability(total_hires, num_of_applicants)
###Output
_____no_output_____
###Markdown
^ this number can be used to tell more human readable calculations such as turning it into a percentage by multiplying it by 100
###Code
def percentage(prob):
percentage = prob * 100
return '{:.4f}% chance of occurence'.format(percentage)
percentage(prob)
###Output
_____no_output_____
###Markdown
To get the fractional form of the probabiility, divide both the divide both $ A $ and $ sample space $ by $ A $, then(if needed), round the denominiator
###Code
def fraction_probability(event_count,sample_space):
denominator = round( sample_space/event_count)
numerator = int(event_count/event_count)
return '{}/{} chance of occurence'.format(numerator, denominator)
fraction_probability(total_hires, num_of_applicants)
###Output
_____no_output_____
###Markdown
Let's plug our numbers from google into these functions to the chances of getting hired
###Code
# probability of being hired
prob_hired = probability(total_hires,num_of_applicants)
display(prob_hired)
# Percentage of people hired
display(percentage_hired(prob_hired))
# Fraction of people hired
display(fraction_probability(total_hires,num_of_applicants))
###Output
_____no_output_____
###Markdown
^Use the formulas above to solve the following questions:1. It rained 3 days last month, what was the probability of it raining? Express this in percentage and fractions as well2. You had 28 days last year where your website had over 100,000 unique visitors. What was the probability of any one day having over 100k visitors? What percentage of days had over 100k visitors?3. Your website crashes every 3rd Tuesday and every 2nd Thursday. What is the probability of a crash occuring in a 31 day month? Express this in percentages and fractions Conditional ProbabilityConditonal probability takes this a bit further in that it gets more descriptive. The conditional probability of an event occurring ( called $ B $ ) is the probability that the event will occur, given the knowledge that another event ( $ A $ ) has already occurred.What this means is that $ B $ happening is dependent on $ A $ happening.Example:> of those 7000 hired, let's say that 1500 of them have brown hair> What are the chances that you were hired AND had brown hair?So our $ sample space $ is still 3 million, the number of applicants hasn't changedOur count of hires $ A $ is still the same at 7000.The counts in our new variable $ B $ are a subset of A ($ A $ must occur for $ B $ to be possible)> You'll see this expressed as:$ B $ is a subset of $ A $ or $B ⊂ A$You'll see this expressed in mathematics as:$ P(A|B) = \frac{P(A and B)}{P(A)} $Let's head to Python to see this in action!
###Code
# To get the conditional probability
def conditional_prob(subset_count, event_count, sample_space):
subset_prob = subset_count/ sample_space
event_prob = event_count / sample_space
conditional_p = (subset_prob)/ event_prob
return 'The conditional probability of this occuring out of {} events is {}'.format(sample_space,conditional_p)
# To get the percentage of the conditional probability
def conditional_prob_percentage(cond_prob):
percentage = cond_prob * 100
return '{}% chance of occuring'.format(percentage)
# To get the fracitonal representation
def conditional_prob_fraction(cond_prob,sample_space):
numerator = round(cond_prob / cond_prob)
denominator = round(sample_space / cond_prob)
return '{} / {} chance of occurence'.format(numerator, denominator)
###Output
_____no_output_____
###Markdown
To really make this stick, were going to plug the numbers from our hiring into the functions!
###Code
# Number of people with black hair is 1500
num_of_brown_hair = 1500
cond_prob = conditional_prob(num_of_brown_hair,total_hires,num_of_applicants)
display(cond_prob)
cond_percent = conditional_prob_percentage(0.0005)
display(cond_percent)
fraction = conditional_prob_fraction(0.0005,num_of_applicants)
display(fraction)
# Given that a passenger survived, what are the odds that they are male?
DATA = "train.csv"
###Output
_____no_output_____ |
_notebooks/2020-12-29-fahonaws.ipynb | ###Markdown
Accessing Folding@Home data on AWS- toc: false- branch: master- badges: true- comments: false- categories: [data science, molecular modeling] Some F@H data is [freely accessible on AWS](https://registry.opendata.aws/foldingathome-covid19/).This will be a relatively short post on accessing and navigating the data on AWS.If you regularly use AWS, this will be nothing new. If you're a grad student who has only ever navigated local file directories or used `scp`/`rsync`/`ssh` to interact with remote clusters, this might be your first time interacting with files on AWS S3.The python environment is fairly straightforward analytical environment, but with s3fs, boto3, and botocore to interact with files on S3`conda create -n fahaws python=3.7 pandas s3fs jupyter ipykernel -c conda-forge -yq`(Active environment)`python -m pip install boto3 botocore` The AWS CLIThe tools to navigate files within AWS directories follow that of unix-like systems.[AWS CLI installation](https://docs.aws.amazon.com/cli/latest/userguide/cli-chap-install.html).`aws s3 ls s3://fah-public-data-covid19-absolute-free-energy/ --no-sign-request` to list files within this particular S3 bucket. The no sign request flag at the end helps us bypass the need for any credentials.You can read from stdout or pipe the output to a textfile, but this will be your bread and butter for wading through terabytes and terabytes of F@H data.As of this post (Dec 2020), looks like the files in `free_energy_data/` have been last updated end of Sept 2020 Summary of free energy results dataFortunately, loading remote files via pandas is a common task, so there are convenient functions.Loading a dataframe over S3 is just like loading a dataframe locally (note the S3 string syntax)The column `febkT` looks like the binding free energies in units of $k_B T$ (multiply by Boltzmann's constant and temperature to get energies in kJ or kcal).It's worth mentioning that the value of the binding free energy is not as helpful as the _relative_ binding free energy to find the best binder of the bunch (how do these free energies compare against each other?)
###Code
import pandas as pd
df = pd.read_pickle("s3://fah-public-data-covid19-absolute-free-energy/free_energy_data/results.pkl")
df.head()
###Output
_____no_output_____
###Markdown
Some code to iterate through these bucketsPythonically, we can build some S3 code to list each object in this S3 bucket.
###Code
import boto3
from botocore import UNSIGNED
from botocore.client import Config
s3 = boto3.resource('s3', config=Config(signature_version=UNSIGNED))
s3_client = boto3.client('s3', config=Config(signature_version=UNSIGNED))
bucket_name = "fah-public-data-covid19-absolute-free-energy"
bucket = s3.Bucket(bucket_name)
###Output
_____no_output_____
###Markdown
This S3 bucket is very large -- all the simulation inputs, trajectories, and outputs are in here, so it will take a while to enumerate every object.Instead, we'll just make a generator and pull out a single item for proof-of-concept.
###Code
paginator = s3_client.get_paginator('list_objects_v2')
pages = paginator.paginate(Bucket=bucket_name)
def page_iterator(pages):
for page in pages:
for item in page['Contents']:
yield item['Key']
all_objects = page_iterator(pages)
next(all_objects)
###Output
_____no_output_____
###Markdown
And if you wanted to, you could layer a filter over the generator to impose some logic like filtering for the top-level directories
###Code
first_level_dirs = filter(lambda x: x.count('/')==1, all_objects)
###Output
_____no_output_____
###Markdown
Unix-like python filesytem libraries[S3FS](https://s3fs.readthedocs.io/en/latest/), built on botocore and fsspec, has a very unix-like syntax to navigate and open files
###Code
import s3fs
fs = s3fs.S3FileSystem(anon=True)
fs.ls(bucket_name)
fs.ls(bucket_name + "/free_energy_data")
with fs.open('fah-public-data-covid19-absolute-free-energy/free_energy_data/hello.txt', 'r') as f:
print(f.read())
with fs.open("fah-public-data-covid19-absolute-free-energy/free_energy_data/organization.pkl", 'rb') as f:
organization_df = pd.read_pickle(f)
organization_df.head()
###Output
_____no_output_____ |
Tutorial2/MNIST_MLP.ipynb | ###Markdown
###Code
import torch
import numpy as np
from torchvision import datasets
import torchvision.transforms as transforms
from torch.utils.data.sampler import SubsetRandomSampler
# number of subprocesses to use for data loading
num_workers = 0
# how many samples per batch to load
batch_size = 20
# percentage of training set to use as validation
valid_size = 0.2
# convert data to torch.FloatTensor
transform = transforms.ToTensor()
# choose the training and testing datasets
train_data = datasets.MNIST(root = 'data', train = True, download = True, transform = transform)
test_data = datasets.MNIST(root = 'data', train = False, download = True, transform = transform)
# obtain training indices that will be used for validation
num_train = len(train_data)
indices = list(range(num_train))
np.random.shuffle(indices)
split = int(np.floor(valid_size * num_train))
train_index, valid_index = indices[split:], indices[:split]
# define samplers for obtaining training and validation batches
train_sampler = SubsetRandomSampler(train_index)
valid_sampler = SubsetRandomSampler(valid_index)
# prepare data loaders
train_loader = torch.utils.data.DataLoader(train_data, batch_size = batch_size,
sampler = train_sampler, num_workers = num_workers)
valid_loader = torch.utils.data.DataLoader(train_data, batch_size = batch_size,
sampler = valid_sampler, num_workers = num_workers)
test_loader = torch.utils.data.DataLoader(test_data, batch_size = batch_size,
num_workers = num_workers)
train_data
import matplotlib.pyplot as plt
%matplotlib inline
# obtain one batch of training images
dataiter = iter(train_loader)
images, labels = dataiter.next()
images = images.numpy()
# plot the images in the batch, along with the corresponding labels
fig = plt.figure(figsize=(25, 4))
for idx in np.arange(20):
ax = fig.add_subplot(2, 20/2, idx+1, xticks=[], yticks=[])
ax.imshow(np.squeeze(images[idx]), cmap='gray')
# print out the correct label for each image
# .item() gets the value contained in a Tensor
ax.set_title(str(labels[idx].item()))
img = np.squeeze(images[1])
fig = plt.figure(figsize = (12,12))
ax = fig.add_subplot(111)
ax.imshow(img, cmap='gray')
width, height = img.shape
thresh = img.max()/2.5
for x in range(width):
for y in range(height):
val = round(img[x][y],2) if img[x][y] !=0 else 0
ax.annotate(str(val), xy=(y,x),
horizontalalignment='center',
verticalalignment='center',
color='white' if img[x][y]<thresh else 'black')
import torch.nn as nn
import torch.nn.functional as F
# define NN architecture
class Net(nn.Module):
def __init__(self):
super(Net,self).__init__()
# number of hidden nodes in each layer (512)
hidden_1 = 512
hidden_2 = 512
# linear layer (784 -> hidden_1)
self.fc1 = nn.Linear(28*28, 512)
# linear layer (n_hidden -> hidden_2)
self.fc2 = nn.Linear(512,512)
self.fc4 = nn.Linear(512,512)
# linear layer (n_hidden -> 10)
self.fc3 = nn.Linear(512,10)
# dropout layer (p=0.2)
# dropout prevents overfitting of data
self.droput = nn.Dropout(0.2)
def forward(self,x):
# flatten image input
x = x.view(-1,28*28)
# add hidden layer, with relu activation function
x = F.relu(self.fc1(x))
# add dropout layer
x = self.droput(x)
# add hidden layer, with relu activation function
x = F.relu(self.fc2(x))
x = F.relu(self.fc4(x))
# add dropout layer
x = self.droput(x)
# add output layer
x = self.fc3(x)
return x
# initialize the NN
model = Net()
print(model)
model.cuda()
# specify loss function (categorical cross-entropy)
criterion = nn.CrossEntropyLoss()
# specify optimizer (stochastic gradient descent) and learning rate = 0.01
optimizer = torch.optim.SGD(model.parameters(),lr = 0.01)
# number of epochs to train the model
n_epochs = 50
# initialize tracker for minimum validation loss
valid_loss_min = np.Inf # set initial "min" to infinity
for epoch in range(n_epochs):
# monitor losses
train_loss = 0
valid_loss = 0
###################
# train the model #
###################
model.train() # prep model for training
for data,label in train_loader:
if torch.cuda.is_available():
data, label = data.cuda(), label.cuda()
# clear the gradients of all optimized variables
optimizer.zero_grad()
# forward pass: compute predicted outputs by passing inputs to the model
output = model(data)
# calculate the loss
loss = criterion(output,label)
# backward pass: compute gradient of the loss with respect to model parameters
loss.backward()
# perform a single optimization step (parameter update)
optimizer.step()
# update running training loss
train_loss += loss.item() * data.size(0)
######################
# validate the model #
######################
model.eval() # prep model for evaluation
for data,label in valid_loader:
if torch.cuda.is_available():
data, label = data.cuda(), label.cuda()
# forward pass: compute predicted outputs by passing inputs to the model
output = model(data)
# calculate the loss
loss = criterion(output,label)
# update running validation loss
valid_loss += loss.item() * data.size(0)
# print training/validation statistics
# calculate average loss over an epoch
train_loss = train_loss / len(train_loader.sampler)
valid_loss = valid_loss / len(valid_loader.sampler)
print('Epoch: {} \tTraining Loss: {:.6f} \tValidation Loss: {:.6f}'.format(
epoch+1,
train_loss,
valid_loss
))
# save model if validation loss has decreased
if valid_loss <= valid_loss_min:
print('Validation loss decreased ({:.6f} --> {:.6f}). Saving model ...'.format(
valid_loss_min,
valid_loss))
torch.save(model.state_dict(), 'model.pt')
valid_loss_min = valid_loss
# initialize lists to monitor test loss and accuracy
test_loss = 0.0
class_correct = list(0. for i in range(10))
class_total = list(0. for i in range(10))
model.eval() # prep model for evaluation
for data, target in test_loader:
if torch.cuda.is_available():
data, target = data.cuda(), target.cuda()
# forward pass: compute predicted outputs by passing inputs to the model
output = model(data)
# calculate the loss
loss = criterion(output, target)
# update test loss
test_loss += loss.item()*data.size(0)
# convert output probabilities to predicted class
_, pred = torch.max(output, 1)
# compare predictions to true label
correct = np.squeeze(pred.eq(target.data.view_as(pred)))
# calculate test accuracy for each object class
for i in range(len(target)):
label = target.data[i]
class_correct[label] += correct[i].item()
class_total[label] += 1
# calculate and print avg test loss
test_loss = test_loss/len(test_loader.sampler)
print('Test Loss: {:.6f}\n'.format(test_loss))
for i in range(10):
if class_total[i] > 0:
print('Test Accuracy of %5s: %2d%% (%2d/%2d)' % (
str(i), 100 * class_correct[i] / class_total[i],
np.sum(class_correct[i]), np.sum(class_total[i])))
else:
print('Test Accuracy of %5s: N/A (no training examples)' % (classes[i]))
print('\nTest Accuracy (Overall): %2d%% (%2d/%2d)' % (
100. * np.sum(class_correct) / np.sum(class_total),
np.sum(class_correct), np.sum(class_total)))
###Output
Test Loss: 0.072597
Test Accuracy of 0: 99% (972/980)
Test Accuracy of 1: 99% (1127/1135)
Test Accuracy of 2: 98% (1012/1032)
Test Accuracy of 3: 98% (991/1010)
Test Accuracy of 4: 97% (962/982)
Test Accuracy of 5: 98% (875/892)
Test Accuracy of 6: 98% (940/958)
Test Accuracy of 7: 98% (1010/1028)
Test Accuracy of 8: 97% (947/974)
Test Accuracy of 9: 97% (984/1009)
Test Accuracy (Overall): 98% (9820/10000)
|
docs/MonsterScrap.ipynb | ###Markdown
MonsterScrap docThese functions allow to perform web scrapping on the Monster platform, to collect job detail.____Requirements:
###Code
import sys
sys.path.append("..")
from Jobtimize.rotateproxies import RotateProxies
from requests import get, Timeout
from requests.exceptions import HTTPError, ProxyError
from concurrent.futures import ThreadPoolExecutor
from itertools import islice
from bs4 import BeautifulSoup
from unicodedata import normalize
from json import loads
import re
###Output
_____no_output_____
###Markdown
The *scrapBody()* function allows to take the body part of an html document from the URL. This is to avoid redundant code in the main function.
###Code
def scrapBody(url, proxy = None):
with get(url, proxies = proxy) as response:
body = BeautifulSoup(response.text, 'html.parser').body
return body
###Output
_____no_output_____
###Markdown
The *idFromLink()* function allows to extract the job ID from link.IDs come in two different forms:- A series of 9 numbers- A string of characters xxxxxxxxxx-xxxx-xxxx-xxxx-xxxxxx-xxxxxxxxxxxxLinks on Monster come in 3 different forms: - Pages generated with ASP including the ID, composed only by 9 digits- The form \*/monster/\* with the ID, composed by string, which is the standard form for the main site- "job offer" *(in the language of the country)* with the ID in figures at the end, which is the standard form for each sub-sites.
###Code
def idFromLink(link):
if ".aspx" in link:
jobID = link[-14:-5]
elif "/monster/" in link:
jobID = re.findall(r'monster/.+?\?', link)[0][8:-1]
else:
jobID = link[link.rfind('/')+1:]
return jobID
###Output
_____no_output_____
###Markdown
The `scrapMonsterID()` function extracts the *jobIDs* for each job and country from the search results provided by Monster.The [monster.co.uk](https://www.monster.co.uk/internationalJobs) site has access to the main master database, unlike the sub-sites which only have access to their own country the database.A query allows to have the total match if it exists. If there are more than 5000, the division *resultCountLabel* displays "5000+". If there is none, the division does not appear on the page.During the page browsing, $p$ greater than 1, the site has a behavior that displays the absence of results on a page $p$ while there are some on the page $p+1$ and therefore ignores the 20 results of the page $p$. The function counts it as an error.The absence of *resultCountLabel* is not interpreted as the end of the results unless the size of the list of jobs covered is equal to (or greater than) the match minus the number of jobs ignored by the error.
###Code
def scrapMonsterID(searchList, countryList, prox = False):
setID = set()
for search in searchList:
search = search.replace(" ","+")
if prox: proxies = RotateProxies()
proxy = None
for country in countryList:
match = 5001
error = 0
listID = set()
page = 1
while True:
url = "https://www.monster.co.uk/medley?q={}&fq=countryabbrev_s%3A{}&pg={}".format(
search, country, page)
if page % 50 == 0 and prox: proxy = proxies.next()
try:
body = scrapBody(url, proxy)
except (Timeout, ProxyError):
if prox:
proxy = proxies.next()
continue
else:
break
except HTTPError:
break
else:
if body.find(id="resultCountLabel") is None:
if len(listID) == 0:
break
else:
error += 1
if len(listID) >= (match - 20 * error):
break
else:
page += 1
continue
else:
match = int(
re.sub(
"\D", "",
body.find(
id="resultCountLabel").text.split()[-1]))
links = [
link.a.attrs['href']
for link in body.find_all("div", class_="jobTitle")
]
listID = {idFromLink(link) for link in links}
page += 1
setID = setID.union(listID)
return setID
###Output
_____no_output_____
###Markdown
The *dicoFromJson()* function normalizes the data of the request response. For a *jobID*, it collects information about the ad, the company and the specificities of the job in a dictionary.- description: long description- country: 2-letter abbreviation of the country- city: full city name- posted: job post creation date- header: post title- company: company name- type: type of employment contract (employee, intern...)- category: job category- url: post url redirection
###Code
def dicoFromJson(args):
jobID, proxy = args
url = "https://job-openings.monster.com/v2/job/pure-json-view?jobid={}".format(
jobID)
try:
query = get(url, proxy).text
except HTTPError:
return {}
dico = loads(
normalize('NFKD', query).encode('ascii', 'ignore'))
general = (("description", "jobDescription"),
("country", "jobLocationCountry"),
("city", "jobLocationCity"),
("posted", "postedDate"))
company = (("header", "companyHeader"),
("company", "name"))
tracks = (("type", "eVar33"),
("category", "eVar28"))
ginfo, cinfo, tinfo = {}, {}, {}
for g in general:
try:
ginfo[g[0]] = normalize(
"NFKD",
" ".join(BeautifulSoup(dico[g[1]], 'lxml').get_text().split()))
except KeyError:
ginfo[g[0]] = ""
for c in company:
try:
cinfo[c[0]] = BeautifulSoup(dico["companyInfo"][c[1]],
'lxml').get_text().rstrip()
except KeyError:
cinfo[c[0]] = ""
for t in tracks:
try:
tinfo[t[0]] = BeautifulSoup(dico["adobeTrackingProperties"][t[1]],
'lxml').get_text().rstrip()
except KeyError:
tinfo[t[0]] = ""
dico = {**ginfo, **cinfo, **tinfo}
dico["url"] = "https://job-openings.monster.co.uk/monster/{}".format(jobID)
return dico
###Output
_____no_output_____
###Markdown
**`MonsterScrap()`** is the main function which collects and standardizes data on the Monster site. Threads are used depending on the size of the results for data normalization.
###Code
def MonsterScrap(searchList, countryList, prox = False):
scraped = list()
setID = scrapMonsterID(searchList, countryList, prox)
if len(setID) < 20:
workers = len(setID)
else:
workers = len(setID) // 5
if prox:
proxies = list(islice(RotateProxies().proxies, workers)) * len(setID)
else:
proxies = [None] * len(setID)
with ThreadPoolExecutor(workers) as executor:
for result in executor.map(dicoFromJson, zip(setID, proxies)):
scraped.append(result)
return scraped
###Output
_____no_output_____
###Markdown
Example of useLet's do research on the data scientist post in the United Kingdom only. Preview the 3rd item in the list.
###Code
listOfJob = MonsterScrap(["Data Scientist"],["UK"])
listOfJob[2]
###Output
_____no_output_____ |
notebooks/RNN-Morse-chars_blk.ipynb | ###Markdown
Model with character recognition - keying recognition based - with blank characterBuilds on `RNN-Morse-chars-feat` and post processes the keying recognition (dits, dahs and silences) to best reveal dits, dots and character and word separators. Then it can be processed by a programmatic logic or another RNN model.Labels include a fictitious "blank" character that masks character labels outside the character spacing period. This follows the labeling done in the [nn-morse](https://github.com/pd0wm/nn-morse) project.Unfortunately this does not work. Create stringEach character in the alphabet should happen a large enough number of times. As a rule of thumb we will take some multiple of the number of characters in the alphabet. If the multiplier is large enough the probability of each character appearance will be even over the alphabet.
###Code
import MorseGen
morse_gen = MorseGen.Morse()
alphabet = morse_gen.alphabet14
print(132/len(alphabet))
morsestr = MorseGen.get_morse_str(nchars=132*5, nwords=27*5, chars=alphabet)
print(alphabet)
print(len(morsestr), morsestr)
###Output
_____no_output_____
###Markdown
Generate dataframe and extract envelope
###Code
Fs = 8000
samples_per_dit = morse_gen.nb_samples_per_dit(Fs, 13)
n_prev = int((samples_per_dit/128)*19) + 1
print(f'Samples per dit at {Fs} Hz is {samples_per_dit}. Decimation is {samples_per_dit/128:.2f}. Look back is {n_prev}.')
label_df = morse_gen.encode_df_decim_blk_str(morsestr, samples_per_dit, 128, alphabet)
env = label_df['env'].to_numpy()
print(type(env), len(env))
import numpy as np
def get_new_data(morse_gen, SNR_dB=-23, nchars=132, nwords=27, phrase=None, alphabet="ABC"):
if not phrase:
phrase = MorseGen.get_morse_str(nchars=nchars, nwords=nwords, chars=alphabet)
print(len(phrase), phrase)
Fs = 8000
samples_per_dit = morse_gen.nb_samples_per_dit(Fs, 13)
n_prev = int((samples_per_dit/128)*19) + 1 # number of samples to look back is longest 3 element ('---' or 'O') + word separator (12+7 = 19)
print(f'Samples per dit at {Fs} Hz is {samples_per_dit}. Decimation is {samples_per_dit/128:.2f}. Look back is {n_prev}.')
label_df = morse_gen.encode_df_decim_blk_str(phrase, samples_per_dit, 128, alphabet)
# extract the envelope
envelope = label_df['env'].to_numpy()
# remove the envelope
label_df.drop(columns=['env'], inplace=True)
SNR_linear = 10.0**(SNR_dB/10.0)
SNR_linear *= 256 # Apply original FFT
print(f'Resulting SNR for original {SNR_dB} dB is {(10.0 * np.log10(SNR_linear)):.2f} dB')
t = np.linspace(0, len(envelope)-1, len(envelope))
power = np.sum(envelope**2)/len(envelope)
noise_power = power/SNR_linear
noise = np.sqrt(noise_power)*np.random.normal(0, 1, len(envelope))
# noise = butter_lowpass_filter(raw_noise, 0.9, 3) # Noise is also filtered in the original setup from audio. This empirically simulates it
signal = envelope + noise
return envelope, signal, label_df, n_prev
###Output
_____no_output_____
###Markdown
Try it...
###Code
import matplotlib.pyplot as plt
envelope, signal, label_df, n_prev = get_new_data(morse_gen, SNR_dB=-17, phrase=morsestr, alphabet=alphabet)
# Show
print(n_prev)
print(type(signal), signal.shape)
print(type(label_df), label_df.shape)
x0 = 0
x1 = 1500
plt.figure(figsize=(50,4+0.5*len(morse_gen.alphabet)))
plt.plot(signal[x0:x1]*0.5, label="sig")
plt.plot(envelope[x0:x1]*0.9, label='env')
plt.plot(label_df[x0:x1].dit*0.9 + 1.0, label='dit')
plt.plot(label_df[x0:x1].dah*0.9 + 1.0, label='dah')
plt.plot(label_df[x0:x1].ele*0.9 + 2.0, label='ele')
plt.plot(label_df[x0:x1].chr*0.9 + 2.0, label='chr')
plt.plot(label_df[x0:x1].wrd*0.9 + 2.0, label='wrd')
plt.plot(label_df[x0:x1].blk*(len(alphabet)+1) + 3.0, label='blk', alpha=0.5)
for i, a in enumerate(alphabet):
plt.plot(label_df[x0:x1][a]*0.9 + 4.0 + i, label=a)
plt.title("signal and labels")
plt.legend()
plt.grid()
###Output
_____no_output_____
###Markdown
Create data loader for keying model Define keying dataset
###Code
import torch
class MorsekeyingDataset(torch.utils.data.Dataset):
def __init__(self, morse_gen, device, SNR_dB=-23, nchars=132, nwords=27, phrase=None, alphabet="ABC"):
self.envelope, self.signal, self.label_df0, self.seq_len = get_new_data(morse_gen, SNR_dB=SNR_dB, phrase=phrase, alphabet=alphabet)
self.label_df = self.label_df0[['dit','dah','ele','chr','wrd']]
self.X = torch.FloatTensor(self.signal).to(device)
self.y = torch.FloatTensor(self.label_df.values).to(device)
def __len__(self):
return self.X.__len__() - self.seq_len
def __getitem__(self, index):
return (self.X[index:index+self.seq_len], self.y[index+self.seq_len])
def get_envelope(self):
return self.envelope
def get_signal(self):
return self.signal
def get_labels(self):
return self.label_df
def get_labels0(self):
return self.label_df0
def get_seq_len(self):
return self.seq_len()
###Output
_____no_output_____
###Markdown
Define keying data loader
###Code
device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
train_keying_dataset = MorsekeyingDataset(morse_gen, device, -23, 132*5, 27*5, morsestr, alphabet)
train_keying_loader = torch.utils.data.DataLoader(train_keying_dataset, batch_size=1, shuffle=False) # Batch size must be 1
signal = train_keying_dataset.get_signal()
label_df = train_keying_dataset.get_labels()
label_df0 = train_keying_dataset.get_labels0()
print(type(signal), signal.shape)
print(type(label_df), label_df.shape)
x0 = 0
x1 = 1500
plt.figure(figsize=(50,3))
plt.plot(signal[x0:x1]*0.5, label="sig")
plt.plot(envelope[x0:x1]*0.9, label='env')
plt.plot(label_df[x0:x1].dit*0.9 + 1.0, label='dit')
plt.plot(label_df[x0:x1].dah*0.9 + 1.0, label='dah')
plt.plot(label_df[x0:x1].ele*0.9 + 2.0, label='ele')
plt.plot(label_df[x0:x1].chr*0.9 + 2.0, label='chr')
plt.plot(label_df[x0:x1].wrd*0.9 + 2.0, label='wrd')
plt.title("keying - signal and labels")
plt.legend()
plt.grid()
###Output
_____no_output_____
###Markdown
Create model classesThe model classes are the same they will be instantiated differently for keying and character models Create model for keying recognition
###Code
import torch
import torch.nn as nn
class MorseLSTM(nn.Module):
"""
Initial implementation
"""
def __init__(self, device, input_size=1, hidden_layer_size=8, output_size=6):
super().__init__()
self.device = device # This is the only way to get things work properly with device
self.hidden_layer_size = hidden_layer_size
self.lstm = nn.LSTM(input_size=input_size, hidden_size=hidden_layer_size)
self.linear = nn.Linear(hidden_layer_size, output_size)
self.hidden_cell = (torch.zeros(1, 1, self.hidden_layer_size).to(self.device),
torch.zeros(1, 1, self.hidden_layer_size).to(self.device))
def forward(self, input_seq):
lstm_out, self.hidden_cell = self.lstm(input_seq.view(len(input_seq), 1, -1), self.hidden_cell)
predictions = self.linear(lstm_out.view(len(input_seq), -1))
return predictions[-1]
def zero_hidden_cell(self):
self.hidden_cell = (
torch.zeros(1, 1, self.hidden_layer_size).to(device),
torch.zeros(1, 1, self.hidden_layer_size).to(device)
)
class MorseBatchedLSTM(nn.Module):
"""
Initial implementation
"""
def __init__(self, device, input_size=1, hidden_layer_size=8, output_size=6):
super().__init__()
self.device = device # This is the only way to get things work properly with device
self.input_size = input_size
self.hidden_layer_size = hidden_layer_size
self.lstm = nn.LSTM(input_size=input_size, hidden_size=hidden_layer_size)
self.linear = nn.Linear(hidden_layer_size, output_size)
self.hidden_cell = (torch.zeros(1, 1, self.hidden_layer_size).to(self.device),
torch.zeros(1, 1, self.hidden_layer_size).to(self.device))
self.m = nn.Softmax(dim=-1)
def forward(self, input_seq):
#print(len(input_seq), input_seq.shape, input_seq.view(-1, 1, 1).shape)
lstm_out, self.hidden_cell = self.lstm(input_seq.view(-1, 1, self.input_size), self.hidden_cell)
predictions = self.linear(lstm_out.view(len(input_seq), -1))
return self.m(predictions[-1])
def zero_hidden_cell(self):
self.hidden_cell = (
torch.zeros(1, 1, self.hidden_layer_size).to(device),
torch.zeros(1, 1, self.hidden_layer_size).to(device)
)
class MorseLSTM2(nn.Module):
"""
LSTM stack
"""
def __init__(self, device, input_size=1, hidden_layer_size=8, output_size=6, dropout=0.2):
super().__init__()
self.device = device # This is the only way to get things work properly with device
self.hidden_layer_size = hidden_layer_size
self.lstm = nn.LSTM(input_size, hidden_layer_size, num_layers=2, dropout=dropout)
self.linear = nn.Linear(hidden_layer_size, output_size)
self.hidden_cell = (torch.zeros(2, 1, self.hidden_layer_size).to(self.device),
torch.zeros(2, 1, self.hidden_layer_size).to(self.device))
def forward(self, input_seq):
lstm_out, self.hidden_cell = self.lstm(input_seq.view(len(input_seq), 1, -1), self.hidden_cell)
predictions = self.linear(lstm_out.view(len(input_seq), -1))
return predictions[-1]
def zero_hidden_cell(self):
self.hidden_cell = (
torch.zeros(2, 1, self.hidden_layer_size).to(device),
torch.zeros(2, 1, self.hidden_layer_size).to(device)
)
class MorseNoHLSTM(nn.Module):
"""
Do not keep hidden cell
"""
def __init__(self, device, input_size=1, hidden_layer_size=8, output_size=6):
super().__init__()
self.device = device # This is the only way to get things work properly with device
self.hidden_layer_size = hidden_layer_size
self.lstm = nn.LSTM(input_size, hidden_layer_size)
self.linear = nn.Linear(hidden_layer_size, output_size)
def forward(self, input_seq):
h0 = torch.zeros(1, 1, self.hidden_layer_size).to(self.device)
c0 = torch.zeros(1, 1, self.hidden_layer_size).to(self.device)
lstm_out, _ = self.lstm(input_seq.view(len(input_seq), 1, -1), (h0, c0))
predictions = self.linear(lstm_out.view(len(input_seq), -1))
return predictions[-1]
class MorseBiLSTM(nn.Module):
"""
Attempt Bidirectional LSTM: does not work
"""
def __init__(self, device, input_size=1, hidden_size=12, num_layers=1, num_classes=6):
super(MorseEnvBiLSTM, self).__init__()
self.device = device # This is the only way to get things work properly with device
self.hidden_size = hidden_size
self.num_layers = num_layers
self.lstm = nn.LSTM(input_size, hidden_size, num_layers, batch_first=True, bidirectional=True)
self.fc = nn.Linear(hidden_size*2, num_classes) # 2 for bidirection
def forward(self, x):
# Set initial states
h0 = torch.zeros(self.num_layers*2, x.size(0), self.hidden_size).to(device) # 2 for bidirection
c0 = torch.zeros(self.num_layers*2, x.size(0), self.hidden_size).to(device)
# Forward propagate LSTM
out, _ = self.lstm(x.view(len(x), 1, -1), (h0, c0)) # out: tensor of shape (batch_size, seq_length, hidden_size*2)
# Decode the hidden state of the last time step
out = self.fc(out[:, -1, :])
return out[-1]
###Output
_____no_output_____
###Markdown
Create the keying model instance and print the details
###Code
morse_key_model = MorseBatchedLSTM(device, hidden_layer_size=7, output_size=5).to(device) # This is the only way to get things work properly with device
morse_key_loss_function = nn.MSELoss()
morse_key_optimizer = torch.optim.Adam(morse_key_model.parameters(), lr=0.001)
print(morse_key_model)
print(morse_key_model.device)
# Input and hidden tensors are not at the same device, found input tensor at cuda:0 and hidden tensor at cpu
for m in morse_key_model.parameters():
print(m.shape, m.device)
X_t = torch.rand(n_prev)
#X_t = torch.tensor([-0.9648, -0.9385, -0.8769, -0.8901, -0.9253, -0.8637, -0.8066, -0.8066, -0.8593, -0.9341, -1.0000, -0.9385])
X_t = X_t.cuda()
print("Input shape", X_t.shape, X_t.view(-1, 1, 1).shape)
print(X_t)
morse_key_model(X_t)
import torchinfo
channels=10
H=n_prev
W=1
torchinfo.summary(morse_key_model, input_size=(channels, H, W))
###Output
_____no_output_____
###Markdown
Train keying model
###Code
it = iter(train_keying_loader)
X, y = next(it)
print(X.reshape(n_prev,1).shape, X[0].shape, y[0].shape)
print(X[0], y[0])
X, y = next(it)
print(X[0], y[0])
%%time
from tqdm.notebook import tqdm
epochs = 2
morse_key_model.train()
for i in range(epochs):
train_losses = []
loop = tqdm(enumerate(train_keying_loader), total=len(train_keying_loader), leave=True)
for j, train in loop:
X_train = train[0][0]
y_train = train[1][0]
morse_key_optimizer.zero_grad()
if morse_key_model.__class__.__name__ in ["MorseLSTM", "MorseLSTM2", "MorseBatchedLSTM", "MorseBatchedLSTM2"]:
morse_key_model.zero_hidden_cell() # this model needs to reset the hidden cell
y_pred = morse_key_model(X_train)
single_loss = morse_key_loss_function(y_pred, y_train)
single_loss.backward()
morse_key_optimizer.step()
train_losses.append(single_loss.item())
# update progress bar
if j % 1000 == 0:
loop.set_description(f"Epoch [{i+1}/{epochs}]")
loop.set_postfix(loss=np.mean(train_losses))
print(f'final: {i+1:3} epochs loss: {np.mean(train_losses):6.4f}')
save_model = True
if save_model:
torch.save(morse_key_model.state_dict(), 'models/morse_key_model')
else:
morse_key_model.load_state_dict(torch.load('models/morse_key_model', map_location=device))
###Output
_____no_output_____
###Markdown
Extract results for next step
###Code
p_key_train = torch.empty(1,5).to(device)
morse_key_model.eval()
loop = tqdm(enumerate(train_keying_loader), total=len(train_keying_loader))
for j, train in loop:
with torch.no_grad():
X_train = train[0]
pred_val = morse_key_model(X_train[0])
p_key_train = torch.cat([p_key_train, pred_val.reshape(1,5)])
# drop first garbage sample
p_key_train = p_key_train[1:]
print(p_key_train.shape)
print(p_key_train[0:2])
p_dits = p_key_train[:,0].to('cpu').numpy()
p_dahs = p_key_train[:,1].to('cpu').numpy()
p_eles = p_key_train[:,2].to('cpu').numpy()
p_chrs = p_key_train[:,3].to('cpu').numpy()
p_wrds = p_key_train[:,4].to('cpu').numpy()
plt.figure(figsize=(50,6))
plt.plot(signal[x0+n_prev:x1+n_prev]*0.5, label="sig")
plt.plot(envelope[x0+n_prev:x1+n_prev]*0.9, label='env')
plt.plot(p_dits[x0:x1]*0.9 + 1.0, label='dit')
plt.plot(p_dahs[x0:x1]*0.9 + 1.0, label='dah')
plt.plot(p_eles[x0:x1]*0.9 + 2.0, label='ele')
plt.plot(p_chrs[x0:x1]*0.9 + 2.0, label='chr')
plt.plot(p_wrds[x0:x1]*0.9 + 2.0, label='wrd')
plt.title("keying - predictions")
plt.legend()
plt.grid()
###Output
_____no_output_____
###Markdown
Post processing
###Code
dit_shift = round(samples_per_dit / 128)
dit2_shift = round(samples_per_dit / 64)
dit3_shift = round(samples_per_dit / 32)
print(dit_shift, dit2_shift, dit3_shift)
dah2_shift = dit2_shift - dit_shift
dah3_shift = dit3_shift - dit2_shift
print(dah2_shift, dah3_shift)
elem_window = p_eles[dit_shift:]
w_dits = p_dits[:-dit_shift] * elem_window
w_dahs = p_dahs[:-dit_shift] * elem_window
w_dahs -= w_dits
w_dahs2 = w_dahs[dah2_shift:]
w_dahs3 = w_dahs[dah3_shift:]
w_dits *= 2.5
w_dits[w_dits > 1.0] = 1.0
label_char_df = train_keying_dataset.get_labels0().drop(columns=['dit','dah','ele','chr','wrd'])
label_char_df = label_char_df[n_prev+dit_shift:].reset_index(drop=True)
plt.figure(figsize=(50,6+0.5*len(alphabet)))
plt.plot(signal[x0+n_prev:x1+n_prev]*0.5, label="sig")
plt.plot(envelope[x0+n_prev:x1+n_prev]*0.9, label='env')
plt.plot(w_dits[x0:x1]*0.9 + 1.0, label='dit')
plt.plot(w_dahs[x0:x1]*0.9 + 1.0, label='dah')
plt.plot(w_dahs2[x0:x1]*0.9 + 1.0, label='da2', alpha=0.5)
plt.plot(w_dahs3[x0:x1]*0.9 + 1.0, label='da3', alpha=0.5)
#plt.plot(p_eles[x0+dit_shift:x1+dit_shift]*0.9 + 2.0, label='ele')
plt.plot(p_chrs[x0+dit_shift:x1+dit_shift]*0.9 + 2.0, label='chr')
plt.plot(p_wrds[x0+dit_shift:x1+dit_shift]*0.9 + 2.0, label='wrd')
for i, a in enumerate(alphabet):
plt.plot(label_char_df[x0:x1][a]*0.45 + 3.0 + i, label=a)
plt.title("keying - predictions - character labels")
plt.legend()
plt.grid()
###Output
_____no_output_____
###Markdown
Bi frequency reconstructionThe idea is to use the resulting dits and dahs sense signals in their original shape. The lengths of dits and dahs are therefore similar. To help distinguish between them by ear one would assign a higher pitch to the dits (thus mimicking the "i" of the dit) and a lower pitch to the dahs (thus mimicking the "ah" of the dah). Moreover to reconstruct the rhythm the dahs sense is delayed by two dits.
###Code
import scipy as sp
import scipy.special
from scipy.io import wavfile
Fdah = 440 # A4
Fdit = 523 # C5
Fs = 8000
noverlap = 128
decim = 128
dit_mod = w_dits[:-dah3_shift]
dit_wav = np.array([[x]*noverlap for x in dit_mod]).flatten()
dah_mod = w_dahs3
dah_wav = np.array([[x]*noverlap for x in dah_mod]).flatten()
dit_wt = (Fdit / Fs)*2*np.pi
dah_wt = (Fdah / Fs)*2*np.pi
dit_tone = np.sin(np.arange(len(dit_wav))*dit_wt)
dah_tone = np.sin(np.arange(len(dah_wav))*dah_wt)
wavfile.write('audio/bif.wav', Fs, dit_tone*dit_wav + dah_tone*dah_wav)
###Output
_____no_output_____
###Markdown
Mono frequency reconstructionHere we stick to more convenient Morse code. In order to do so 2 delayed copies of the dahs sense signal are summed to reconstruct the length of an original dah
###Code
Fcode = 523
mod_len = min(len(w_dits), len(w_dahs), len(w_dahs2), len(w_dahs3))
dit_mod = w_dits[:mod_len]
dah_mod = w_dahs[:mod_len] + w_dahs2[:mod_len] + w_dahs3[:mod_len]
all_mod = dit_mod + dah_mod
mod_wav = np.array([[x]*noverlap for x in all_mod]).flatten()
wt = (Fcode / Fs)*2*np.pi
tone = np.sin(np.arange(len(dit_wav))*wt)
wavfile.write('audio/mof.wav', Fs, tone*mod_wav)
plt.figure(figsize=(50,6))
plt.plot(signal[x0+n_prev:x1+n_prev]*0.5, label="sig")
plt.plot(envelope[x0+n_prev:x1+n_prev]*0.9, label='env')
plt.plot(all_mod[x0:x1]*0.9 + 1.0, label='mod')
plt.title("envelope reconstruction")
plt.legend()
plt.grid()
###Output
_____no_output_____
###Markdown
Construct datasets for character model`label_char_df` are already the correct labels
###Code
t0 = torch.FloatTensor(w_dits).reshape(len(w_dits),1)
t1 = torch.FloatTensor(w_dahs).reshape(len(w_dahs),1)
t2 = torch.FloatTensor(p_chrs[dit_shift:]).reshape(len(w_dahs),1)
t3 = torch.FloatTensor(p_wrds[dit_shift:]).reshape(len(w_dahs),1)
p_key_train = torch.cat([t0,t1,t2,t3], axis=1)
p_key_train.shape
###Output
_____no_output_____
###Markdown
Create data loader for character model Define character dataset
###Code
class MorseCharacterDataset(torch.utils.data.Dataset):
def __init__(self, key_train, label_df, seq_len):
self.label_df = label_df
self.X = key_train.to(device)
self.y = torch.FloatTensor(self.label_df.values).to(device)
self.seq_len = seq_len
def __len__(self):
return self.X.__len__() - self.seq_len
def __getitem__(self, index):
return (self.X[index:index+self.seq_len], self.y[index+self.seq_len])
def get_X(self):
return self.X
def get_labels(self):
return self.label_df
def get_seq_len(self):
return self.seq_len
###Output
_____no_output_____
###Markdown
Define character data loader
###Code
train_character_dataset = MorseCharacterDataset(p_key_train[dit_shift:], label_char_df, n_prev)
train_character_loader = torch.utils.data.DataLoader(train_character_dataset, batch_size=1, shuffle=False) # Batch size must be 1
X_train_chr = train_character_dataset.get_X().cpu()
label_df_chr = train_character_dataset.get_labels()
print(type(X_train_chr), X_train_chr.shape)
print(type(label_df_chr), label_df_chr.shape)
x0 = 0
x1 = 1500
plt.figure(figsize=(50,4+0.5*len(alphabet)))
plt.plot(X_train_chr[x0:x1,0], label='dit')
plt.plot(X_train_chr[x0:x1,1], label='dah')
plt.plot(X_train_chr[x0:x1,2] + 1.0, label='chr')
plt.plot(X_train_chr[x0:x1,3] + 1.0, label='wrd')
plt.plot(label_df_chr[x0:x1]['blk']*(len(alphabet)+1) + 2.0, label='blk', alpha=0.5)
for i, a in enumerate(alphabet):
plt.plot(label_df_chr[x0:x1][a]*0.9 + 3.0 + i, label=a)
plt.title("character - signal and labels")
plt.legend()
plt.grid()
###Output
_____no_output_____
###Markdown
Create model for character recognition
###Code
morse_chr_model = MorseBatchedLSTM(device, input_size=4, hidden_layer_size=len(alphabet), output_size=len(alphabet)+1).to(device) # This is the only way to get things work properly with device
morse_chr_loss_function = nn.MSELoss()
morse_chr_optimizer = torch.optim.Adam(morse_chr_model.parameters(), lr=0.001)
print(morse_chr_model)
print(morse_chr_model.device)
# Input and hidden tensors are not at the same device, found input tensor at cuda:0 and hidden tensor at cpu
for m in morse_chr_model.parameters():
print(m.shape, m.device)
X_t = torch.rand(n_prev, 4)
#X_t = torch.tensor([-0.9648, -0.9385, -0.8769, -0.8901, -0.9253, -0.8637, -0.8066, -0.8066, -0.8593, -0.9341, -1.0000, -0.9385])
X_t = X_t.cuda()
print("Input shape", X_t.shape, X_t.view(-1, 1, 4).shape)
morse_chr_model(X_t)
# Does not work...
channels=10
H=n_prev
W=4
torchinfo.summary(morse_chr_model, input_size=(channels, H, W))
###Output
_____no_output_____
###Markdown
Train character model
###Code
it = iter(train_character_loader)
X, y = next(it)
print(X.reshape(n_prev,4).shape, X[0].shape, y[0].shape)
print(y[0])
epochs = 1
morse_chr_model.train()
for i in range(epochs):
train_losses = []
loop = tqdm(enumerate(train_character_loader), total=len(train_character_loader), leave=True)
for j, train in loop:
X_train = train[0][0]
y_train = train[1][0]
morse_chr_optimizer.zero_grad()
if morse_chr_model.__class__.__name__ in ["MorseLSTM", "MorseLSTM2", "MorseBatchedLSTM", "MorseBatchedLSTM2"]:
morse_chr_model.zero_hidden_cell() # this model needs to reset the hidden cell
y_pred = morse_chr_model(X_train)
single_loss = morse_chr_loss_function(y_pred, y_train)
single_loss.backward()
morse_chr_optimizer.step()
train_losses.append(single_loss.item())
# update progress bar
if j % 1000 == 0:
loop.set_description(f"Epoch [{i+1}/{epochs}]")
loop.set_postfix(loss=np.mean(train_losses))
print(f'final: {i+1:3} epochs loss: {np.mean(train_losses):6.4f}')
%%time
p_alpha = {}
for a in alphabet:
p_alpha[a] = []
morse_chr_model.eval()
loop = tqdm(enumerate(train_character_loader), total=len(train_character_loader))
for j, train in loop:
with torch.no_grad():
X_chr = train[0][0]
pred_val = morse_chr_model(X_chr).cpu()
alpha_val = pred_val[1:]
for i, a in enumerate(alphabet):
p_alpha[a].append(alpha_val[i].item()*10.0)
for a in alphabet:
p_alpha[a] = np.array(p_alpha[a])
l_alpha = label_df_chr[n_prev:].reset_index(drop=True)
plt.figure(figsize=(50,3+0.5*len(morse_gen.alphabet)))
plt.plot(X_train_chr[x0+n_prev:x1+n_prev, 0], label='dit')
plt.plot(X_train_chr[x0+n_prev:x1+n_prev, 1], label='dah')
plt.plot(X_train_chr[x0+n_prev:x1+n_prev, 2] + 1.0, label='chr')
plt.plot(X_train_chr[x0+n_prev:x1+n_prev, 3] + 1.0, label='wrd')
for i, a in enumerate(alphabet):
plt.plot(p_alpha[a][x0:x1]*0.9 + 2.0 + i, label=a+"p")
plt.plot(l_alpha[a][x0:x1]*0.9 + 2.0 + i, label=a+"l")
plt.title("predictions")
plt.legend()
plt.grid()
###Output
_____no_output_____ |
Day_63_HW.ipynb | ###Markdown
作業 * 請參閱範例中的 hidden Layer 寫法, 完成 output Layer 的程式
###Code
loss_arr = np.array([])
for i in range(epochs):
# forward pass
z1 = X.dot(input_hidden_weight) + input_hidden_bias
hidden_output = sigmoid(z1)
z2 = hidden_output.dot(hidden_output_weight) + hidden_output_bias
output_value = sigmoid(z2)
# backword pass
loss = (2/X_sample_size)*np.sum(np.abs(y - output_value)) # parital loss respect y
loss_arr = np.append(loss_arr, loss)
partial_output_respect_z2 = derivatives_sigmoid(output_value) # Calculate parital output value respect z2
partial_hidden_output_respect_z1 = derivatives_sigmoid(hidden_output) # Calculate parital hidden output respect z1
partial_loss_respect_z2 = partial_output_respect_z2 * loss
partial_loss_respect_hidden_output = partial_loss_respect_z2.dot(hidden_output_weight.T)
partial_loss_respect_z1 = partial_loss_respect_hidden_output * partial_hidden_output_respect_z1
input_hidden_weight_gradient = X.T.dot(partial_loss_respect_z1)
hidden_output_weight_gradient = hidden_output.T.dot(partial_loss_respect_z2)
input_hidden_weight += input_hidden_weight_gradient * lr
input_hidden_bias += np.sum(partial_loss_respect_z1, axis=0, keepdims=True)
hidden_output_weight += hidden_output_weight_gradient *lr
hidden_output_bias += np.sum(partial_loss_respect_z2, axis=0, keepdims=True)
if i % 100 == 0:
print('epochs: {}, loss: {}'.format(i, loss))
plt.plot(list(range(epochs)), loss_arr)
plt.xlabel('Number of epochs')
plt.ylabel('Loss')
plt.show()
###Output
_____no_output_____ |
docs/Jupyter/VCS_Principles.ipynb | ###Markdown
Visualization Control System Principles[Table Of Content](toc) * [Introduction](intro) * [What](what) * [How](how) * [one dimensional](1d) * [boxfill](box) * [isoline](isol) * [isofill](isof) * [streamlines](stream) * [meshfill](mesh) * [vectors](vec) * [taylor diagrams](taylor) * [Where](where) * [Secondary Objects](secondary) * [Text Objects](text) * [Line/Polygon Objects](line) * [Filled Polygons Objects](fillarea) * [Marker Objects](marker) * [Colormaps](colormap) * [Projections](projection)
###Code
# VCS Objects definitions
import vcs
import cdms2
import os
vcs.download_sample_data_files()
with cdms2.open(os.path.join(vcs.sample_data,"clt.nc")) as f:
clt = f("clt")
u = f("u")
v = f("v")
with cdms2.open(os.path.join(vcs.sample_data,"sampleCurveGrid4.nc")) as f:
curv = f("sample")
with cdms2.open(os.path.join(vcs.sample_data,"sampleGenGrid3.nc")) as f:
gen = f("sample")
x = vcs.init(geometry=(600,400),bg=True)
# Styling for notebook
from IPython.core.display import HTML
HTML("""
<style>
.output_png {
display: table-cell;
text-align: center;
vertical-align: middle;
}
</style>
""")
###Output
Downloading: 'th_yr.nc' from 'https://uvcdat.llnl.gov/cdat/sample_data/' in: /Users/doutriaux1/anaconda2/envs/cdat8/share/uvcdat/sample_data/th_yr.nc
Downloading: 'th_yr.nc' from 'https://uvcdat.llnl.gov/cdat/sample_data/' in: /Users/doutriaux1/anaconda2/envs/cdat8/share/uvcdat/sample_data/th_yr.nc
Downloading: 'th_yr.nc' from 'https://uvcdat.llnl.gov/cdat/sample_data/' in: /Users/doutriaux1/anaconda2/envs/cdat8/share/uvcdat/sample_data/th_yr.nc
###Markdown
IntroductionVCS Allows scientists to produce highly customized plots. Everything can be precisely and logically controlled, without any *guessing* gameEssentially a vcs plot can be broken down into three parts**WHAT** is plotted (e.g data and labels) **HOW** it is rendered (isolines, boxfill, isofill, vectors, etc...) and **WHERE** (location on the page each elements is to be plotted) WhatThis is the scientific piece of information that the user is trying to represent for others (or self) to understand. It can be as raw as a simple numpy object. But it is recommended to use [CDMS](https://github.com/uv-cdat/cdms)'s transient variables. CDNS transient variables contain metadata such as name, units, geospatial information, that can be used by VCS to represent data better.The [tutorials] section has many documents for CDMS. The CDMS documentation can be found [here](http://readthedocs.org/projects/cdms/) HowThis describe the data representation, at the highest level it is a `graphics method` i.e *boxfill*, *isofill*, *vectors*, *streamlines*, *line plot*, etc... But it also contains information to further control these plot types, e.g which colors to use, which levels, lines thickness, etc... Graphic methods also describe how axes and labels show be represented (e.g which axes values to show and which text to use for it, the user might want to show the `-20.` longitude represented as `20S` or the date `2020-01-15` shown as `Jan 2020` Currently VCS supports the following graphic methods: BoxfillBoxfill is used to represent 2 dimensional arrays, filling each array cell with a color representing its value. In the case of rectilinear grids (x and y axes can be representing by a 1 dimension array) represented via CDMS, we use the axes **bounds** to determine the extends of each cell. This is especially useful if an axis is not increasing constantly (e.g, gaussian grid, pressure levels)For more information on boxfill please see the [dedicated tutorial](https://cdat.llnl.gov/Jupyter/boxfill/boxfill.html).
###Code
gm = vcs.createboxfill()
x.plot(clt, gm)
###Output
/Users/doutriaux1/anaconda2/envs/cdat8/lib/python2.7/site-packages/vtk/util/numpy_support.py:135: FutureWarning: Conversion of the second argument of issubdtype from `complex` to `np.complexfloating` is deprecated. In future, it will be treated as `np.complex128 == np.dtype(complex).type`.
assert not numpy.issubdtype(z.dtype, complex), \
###Markdown
IsolineIsoline is a line on a map, chart, or graph connecting points of equal value.For more information on isolines please see the [dedicated tutorial]().
###Code
gm = vcs.createisoline()
x.clear()
x.plot(clt,gm)
###Output
_____no_output_____
###Markdown
IsofillIsofill is similar to isolines (and usually plotted in conjounction with it) except that the are between two consecutive isoline is filled with a color representing the range of values in this area.For more information on boxfill please see the [dedicated tutorial]().
###Code
x.clear()
gm = vcs.createisofill()
x.plot(clt,gm)
###Output
_____no_output_____
###Markdown
MeshfillMeshfill is very similar to boxfill, but is used to represent data on generic grids (a.k.a native representation). Based on the input data and a *mesh*For more information on meshfill please see the [dedicated tutorial]().
###Code
x.clear()
gm = x.createmeshfill()
gm.mesh = True
x.plot(gen, gm)
###Output
_____no_output_____
###Markdown
StreamlinesFor more information on streamlines please see the [dedicated tutorial](https://cdat.llnl.gov/Jupyter/streamlines/streamlines.html).
###Code
x.clear()
gm = vcs.createstreamline()
x.plot(u,v,gm)
###Output
_____no_output_____
###Markdown
Vector PlotsVector plot are a collection of arrows with a given magnitude and direction, each attached to a point in the plane.For more information on streamlines please see the [dedicated tutorial]().
###Code
x.clear()
gm = vcs.createvector()
x.plot(u,v, gm)
###Output
_____no_output_____
###Markdown
Line(1D) PlotsA graph that shows frequency of data along a number line.For more information on 1D please see the [dedicated tutorial](). Also of interest are the [EzPlot Addons](https://github.com/CDAT/vcsaddons/blob/master/EzPlot/Doc/Jupyter/EzPlot%20Spaghetti%20Example.ipynb).
###Code
x.clear()
gm = vcs.create1d()
x.plot(clt[:,34,23]) # extract time serie at one point and plot in 1D
###Output
_____no_output_____
###Markdown
Taylor DiagramsTaylor diagrams are mathematical diagrams designed to graphically indicate which of several approximate representations (or models) of a system, process, or phenomenon is most realistic. This diagram, invented by Karl E. Taylor in 1994 (published in 2001) facilitates the comparative assessment of different models. It is used to quantify the degree of correspondence between the modeled and observed behavior in terms of three statistics: the Pearson correlation coefficient, the root-mean-square error (RMSE) error, and the standard deviation. Taylor diagrams have widely been used to evaluate models designed to study climate and other aspects of Earth’s environment. [See [Wiki](https://en.wikipedia.org/wiki/Taylor_diagramcite_note-8) and [Taylor (2001)](http://onlinelibrary.wiley.com/doi/10.1029/2000JD900719/abstract) for details]Reference: [Taylor, K. E. 2001: Summarizing multiple aspects of model performance in a single diagram. Journal of Geophysical Research, 106(D7): 7183-7192](http://onlinelibrary.wiley.com/doi/10.1029/2000JD900719/abstract)For more detailed information on Taylor Diagrams see this [dedicated tutorial](https://cdat.llnl.gov/Jupyter/Taylor_Diagrams/Taylor_Diagrams.html).
###Code
corr = [.2, .5, .7, .85, .9, .95, .99]
std = [1.6, 1.7, 1.5, 1.2 , .8, .9, .98]
data = cdms2.MV2.array(zip(std, corr))
gm = vcs.createtaylordiagram()
x.clear()
x.plot(data,gm)
###Output
_____no_output_____
###Markdown
WhereThis is the most complicated part of VCS but also one of the most powerful. This controls precisely the location of every component on the plot, these *control* objects are called `templates`. Templates also contain one exception to the WHAT/HOW./WHERE rule as they control texts information, albeit via [primary](primary) objects. Bringing it all together Secondary Objects Positioning Secondary objectsSecondary object positioning is based on a double system.The most basic is called **world coordinate** by changing a secondary object's `worldcoordinate` attribute you can control the coordinates of the rectangle arrea within which the object will be plottted.All coordinate (`.x` and `.y`) are relative to the worldcoordinate attribute (defautling to 0->1)Any coordinate/segment extending beyond the `viewport` rectangle is croppedSee figure bellow for a visual explanation, along with the [vcs script](viewport_and_worldcoordinate.py) to generate it: Text ObjectsText object allow you insert text anywhere on the plotText object are made by combining two different secondary object: text orientation objects and text table objectsFor more details on text in vcs see this [dedicated tutorial]().
###Code
x.clear()
txt = vcs.createtext()
txt.string="A Text Object"
txt.height=25
txt.x = [.5]
txt.y=[.5]
txt.list()
x.plot(txt)
###Output
---------- Text combined (Tc) member (attribute) listings ----------
secondary method = Tc
---------- Text Table (Tt) member (attribute) listings ----------
Tt_name = __texttable_234945596800910
font = 1
spacing = 2
expansion = 100
color = [0.0, 0.0, 0.0, 100.0]
fillincolor = 0
priority = 1
string = ['A Text Object']
viewport = [0.0, 1.0, 0.0, 1.0]
worldcoordinate = [0.0, 1.0, 0.0, 1.0]
x = [0.5]
y = [0.5]
projection = default
---------- Text Orientation (To) member (attribute) listings ----------
To_name = __textorientation_24296950101449
height = 25
angle = 0
path = right
halign = left
valign = half
###Markdown
Line/Polygon ObjectsLine object allow you to draw lines on the plot. By closing the line you can draw a polygonFor more details on line in vcs see this [dedicated tutorial]().
###Code
x.clear()
line = vcs.createline()
line.x = [0.1, .5, 0.9]
line.y = [0.1, .2, 0.9]
x.plot(line)
###Output
_____no_output_____
###Markdown
Filled Polygons ObjectsAllows you to draw a filled polygon on the plotFor more details on filled polygons in vcs see this [dedicated tutorial]().
###Code
x.clear()
filled = vcs.createfillarea()
filled.x = [0.1, .5, 0.9]
filled.y = [0.1, .2, 0.9]
x.plot(filled)
###Output
_____no_output_____
###Markdown
Marker ObjectsAllows you to draw a/many markers on a plot
###Code
x.clear()
mrk = vcs.createmarker()
mrk.type = "hurricane"
mrk.x = [.5]
mrk.y = [.5]
mrk.size = 15
x.plot(mrk)
###Output
_____no_output_____
###Markdown
Colormap Objectscolormap object objects are used to control the colors on vcs plots, they can be attached to secondary object, graphic methods or canvases.Values are in red, green, blue, opacity percents (0 to 100)For more detail see this [dedicated tutorial](https://cdat.llnl.gov/Jupyter/Colormap_Create/Color_map_create_own.html).
###Code
cmap = vcs.createcolormap()
cmap.setcolorcell(0,100,0,0,50) # 50% transparent red color
###Output
_____no_output_____
###Markdown
Projection Objectswhen plotting lat/lon plots (2d graphic methods) you can specifiy and control the projection associated with it. Projection object are then attached to the graphic methodFor more detail see this [dedicated tutorial]().
###Code
x.clear()
gm = vcs.createisofill()
proj = vcs.createprojection()
proj.type="lambert"
proj.list()
proj.originlatitude=30.
gm.projection = proj
x.plot(clt(latitude=(10,50),longitude=(-130,-70)), gm)
###Output
---------- Projection (Proj) member (attribute) listings ----------
('secondary method =', 'Proj')
('name =', '__projection_508306013994393')
('type =', 'lambert conformal c')
('smajor', '=', 1e+20)
('sminor', '=', 1e+20)
('standardparallel1', '=', 1e+20)
('standardparallel2', '=', 1e+20)
('centralmeridian', '=', 1e+20)
('originlatitude', '=', 1e+20)
('falseeasting', '=', 1e+20)
('falsenorthing', '=', 1e+20)
|
caffe_demeshnet/examples/00-classification.ipynb | ###Markdown
Classification: Instant Recognition with CaffeIn this example we'll classify an image with the bundled CaffeNet model (which is based on the network architecture of Krizhevsky et al. for ImageNet).We'll compare CPU and GPU modes and then dig into the model to inspect features and the output. 1. Setup* First, set up Python, `numpy`, and `matplotlib`.
###Code
# set up Python environment: numpy for numerical routines, and matplotlib for plotting
import numpy as np
import matplotlib.pyplot as plt
# display plots in this notebook
%matplotlib inline
# set display defaults
plt.rcParams['figure.figsize'] = (10, 10) # large images
plt.rcParams['image.interpolation'] = 'nearest' # don't interpolate: show square pixels
plt.rcParams['image.cmap'] = 'gray' # use grayscale output rather than a (potentially misleading) color heatmap
###Output
_____no_output_____
###Markdown
* Load `caffe`.
###Code
# The caffe module needs to be on the Python path;
# we'll add it here explicitly.
import sys
caffe_root = '../' # this file should be run from {caffe_root}/examples (otherwise change this line)
sys.path.insert(0, caffe_root + 'python')
import caffe
# If you get "No module named _caffe", either you have not built pycaffe or you have the wrong path.
###Output
_____no_output_____
###Markdown
* If needed, download the reference model ("CaffeNet", a variant of AlexNet).
###Code
import os
if os.path.isfile(caffe_root + 'models/bvlc_reference_caffenet/bvlc_reference_caffenet.caffemodel'):
print 'CaffeNet found.'
else:
print 'Downloading pre-trained CaffeNet model...'
!../scripts/download_model_binary.py ../models/bvlc_reference_caffenet
###Output
Downloading pre-trained CaffeNet model...
...13%, 30 MB, 1100 KB/s, 28 seconds passed
###Markdown
2. Load net and set up input preprocessing* Set Caffe to CPU mode and load the net from disk.
###Code
caffe.set_mode_cpu()
model_def = caffe_root + 'models/bvlc_reference_caffenet/deploy.prototxt'
model_weights = caffe_root + 'models/bvlc_reference_caffenet/bvlc_reference_caffenet.caffemodel'
net = caffe.Net(model_def, # defines the structure of the model
model_weights, # contains the trained weights
caffe.TEST) # use test mode (e.g., don't perform dropout)
###Output
_____no_output_____
###Markdown
* Set up input preprocessing. (We'll use Caffe's `caffe.io.Transformer` to do this, but this step is independent of other parts of Caffe, so any custom preprocessing code may be used). Our default CaffeNet is configured to take images in BGR format. Values are expected to start in the range [0, 255] and then have the mean ImageNet pixel value subtracted from them. In addition, the channel dimension is expected as the first (_outermost_) dimension. As matplotlib will load images with values in the range [0, 1] in RGB format with the channel as the _innermost_ dimension, we are arranging for the needed transformations here.
###Code
# load the mean ImageNet image (as distributed with Caffe) for subtraction
mu = np.load(caffe_root + 'python/caffe/imagenet/ilsvrc_2012_mean.npy')
mu = mu.mean(1).mean(1) # average over pixels to obtain the mean (BGR) pixel values
print 'mean-subtracted values:', zip('BGR', mu)
# create transformer for the input called 'data'
transformer = caffe.io.Transformer({'data': net.blobs['data'].data.shape})
transformer.set_transpose('data', (2,0,1)) # move image channels to outermost dimension
transformer.set_mean('data', mu) # subtract the dataset-mean value in each channel
transformer.set_raw_scale('data', 255) # rescale from [0, 1] to [0, 255]
transformer.set_channel_swap('data', (2,1,0)) # swap channels from RGB to BGR
###Output
_____no_output_____
###Markdown
3. CPU classification* Now we're ready to perform classification. Even though we'll only classify one image, we'll set a batch size of 50 to demonstrate batching.
###Code
# set the size of the input (we can skip this if we're happy
# with the default; we can also change it later, e.g., for different batch sizes)
net.blobs['data'].reshape(50, # batch size
3, # 3-channel (BGR) images
227, 227) # image size is 227x227
###Output
_____no_output_____
###Markdown
* Load an image (that comes with Caffe) and perform the preprocessing we've set up.
###Code
image = caffe.io.load_image(caffe_root + 'examples/images/cat.jpg')
transformed_image = transformer.preprocess('data', image)
plt.imshow(image)
###Output
_____no_output_____
###Markdown
* Adorable! Let's classify it!
###Code
# copy the image data into the memory allocated for the net
net.blobs['data'].data[...] = transformed_image
### perform classification
output = net.forward()
output_prob = output['prob'][0] # the output probability vector for the first image in the batch
print 'predicted class is:', output_prob.argmax()
###Output
_____no_output_____
###Markdown
* The net gives us a vector of probabilities; the most probable class was the 281st one. But is that correct? Let's check the ImageNet labels...
###Code
# load ImageNet labels
labels_file = caffe_root + 'data/ilsvrc12/synset_words.txt'
if not os.path.exists(labels_file):
!../data/ilsvrc12/get_ilsvrc_aux.sh
labels = np.loadtxt(labels_file, str, delimiter='\t')
print 'output label:', labels[output_prob.argmax()]
###Output
_____no_output_____
###Markdown
* "Tabby cat" is correct! But let's also look at other top (but less confident predictions).
###Code
# sort top five predictions from softmax output
top_inds = output_prob.argsort()[::-1][:5] # reverse sort and take five largest items
print 'probabilities and labels:'
zip(output_prob[top_inds], labels[top_inds])
###Output
_____no_output_____
###Markdown
* We see that less confident predictions are sensible. 4. Switching to GPU mode* Let's see how long classification took, and compare it to GPU mode.
###Code
%timeit net.forward()
###Output
_____no_output_____
###Markdown
* That's a while, even for a batch of 50 images. Let's switch to GPU mode.
###Code
caffe.set_device(0) # if we have multiple GPUs, pick the first one
caffe.set_mode_gpu()
net.forward() # run once before timing to set up memory
%timeit net.forward()
###Output
_____no_output_____
###Markdown
* That should be much faster! 5. Examining intermediate output* A net is not just a black box; let's take a look at some of the parameters and intermediate activations.First we'll see how to read out the structure of the net in terms of activation and parameter shapes.* For each layer, let's look at the activation shapes, which typically have the form `(batch_size, channel_dim, height, width)`. The activations are exposed as an `OrderedDict`, `net.blobs`.
###Code
# for each layer, show the output shape
for layer_name, blob in net.blobs.iteritems():
print layer_name + '\t' + str(blob.data.shape)
###Output
_____no_output_____
###Markdown
* Now look at the parameter shapes. The parameters are exposed as another `OrderedDict`, `net.params`. We need to index the resulting values with either `[0]` for weights or `[1]` for biases. The param shapes typically have the form `(output_channels, input_channels, filter_height, filter_width)` (for the weights) and the 1-dimensional shape `(output_channels,)` (for the biases).
###Code
for layer_name, param in net.params.iteritems():
print layer_name + '\t' + str(param[0].data.shape), str(param[1].data.shape)
###Output
_____no_output_____
###Markdown
* Since we're dealing with four-dimensional data here, we'll define a helper function for visualizing sets of rectangular heatmaps.
###Code
def vis_square(data):
"""Take an array of shape (n, height, width) or (n, height, width, 3)
and visualize each (height, width) thing in a grid of size approx. sqrt(n) by sqrt(n)"""
# normalize data for display
data = (data - data.min()) / (data.max() - data.min())
# force the number of filters to be square
n = int(np.ceil(np.sqrt(data.shape[0])))
padding = (((0, n ** 2 - data.shape[0]),
(0, 1), (0, 1)) # add some space between filters
+ ((0, 0),) * (data.ndim - 3)) # don't pad the last dimension (if there is one)
data = np.pad(data, padding, mode='constant', constant_values=1) # pad with ones (white)
# tile the filters into an image
data = data.reshape((n, n) + data.shape[1:]).transpose((0, 2, 1, 3) + tuple(range(4, data.ndim + 1)))
data = data.reshape((n * data.shape[1], n * data.shape[3]) + data.shape[4:])
plt.imshow(data); plt.axis('off')
###Output
_____no_output_____
###Markdown
* First we'll look at the first layer filters, `conv1`
###Code
# the parameters are a list of [weights, biases]
filters = net.params['conv1'][0].data
vis_square(filters.transpose(0, 2, 3, 1))
###Output
_____no_output_____
###Markdown
* The first layer output, `conv1` (rectified responses of the filters above, first 36 only)
###Code
feat = net.blobs['conv1'].data[0, :36]
vis_square(feat)
###Output
_____no_output_____
###Markdown
* The fifth layer after pooling, `pool5`
###Code
feat = net.blobs['pool5'].data[0]
vis_square(feat)
###Output
_____no_output_____
###Markdown
* The first fully connected layer, `fc6` (rectified) We show the output values and the histogram of the positive values
###Code
feat = net.blobs['fc6'].data[0]
plt.subplot(2, 1, 1)
plt.plot(feat.flat)
plt.subplot(2, 1, 2)
_ = plt.hist(feat.flat[feat.flat > 0], bins=100)
###Output
_____no_output_____
###Markdown
* The final probability output, `prob`
###Code
feat = net.blobs['prob'].data[0]
plt.figure(figsize=(15, 3))
plt.plot(feat.flat)
###Output
_____no_output_____
###Markdown
Note the cluster of strong predictions; the labels are sorted semantically. The top peaks correspond to the top predicted labels, as shown above. 6. Try your own imageNow we'll grab an image from the web and classify it using the steps above.* Try setting `my_image_url` to any JPEG image URL.
###Code
# download an image
my_image_url = "..." # paste your URL here
# for example:
# my_image_url = "https://upload.wikimedia.org/wikipedia/commons/b/be/Orang_Utan%2C_Semenggok_Forest_Reserve%2C_Sarawak%2C_Borneo%2C_Malaysia.JPG"
!wget -O image.jpg $my_image_url
# transform it and copy it into the net
image = caffe.io.load_image('image.jpg')
net.blobs['data'].data[...] = transformer.preprocess('data', image)
# perform classification
net.forward()
# obtain the output probabilities
output_prob = net.blobs['prob'].data[0]
# sort top five predictions from softmax output
top_inds = output_prob.argsort()[::-1][:5]
plt.imshow(image)
print 'probabilities and labels:'
zip(output_prob[top_inds], labels[top_inds])
###Output
_____no_output_____ |
tv-script-generation/AB_dlnd_tv_script_generation.ipynb | ###Markdown
TV Script GenerationIn this project, you'll generate your own [Simpsons](https://en.wikipedia.org/wiki/The_Simpsons) TV scripts using RNNs. You'll be using part of the [Simpsons dataset](https://www.kaggle.com/wcukierski/the-simpsons-by-the-data) of scripts from 27 seasons. The Neural Network you'll build will generate a new TV script for a scene at [Moe's Tavern](https://simpsonswiki.com/wiki/Moe's_Tavern). Get the DataThe data is already provided for you. You'll be using a subset of the original dataset. It consists of only the scenes in Moe's Tavern. This doesn't include other versions of the tavern, like "Moe's Cavern", "Flaming Moe's", "Uncle Moe's Family Feed-Bag", etc.. Andrew Burrussedit: May 8, 2018
###Code
"""
DON'T MODIFY ANYTHING IN THIS CELL
"""
import helper
data_dir = './data/simpsons/moes_tavern_lines.txt'
text = helper.load_data(data_dir)
# Ignore notice, since we don't use it for analysing the data
text = text[81:]
###Output
_____no_output_____
###Markdown
Explore the DataPlay around with `view_sentence_range` to view different parts of the data.
###Code
view_sentence_range = (0, 10)
"""
DON'T MODIFY ANYTHING IN THIS CELL
"""
import numpy as np
print('Dataset Stats')
print('Roughly the number of unique words: {}'.format(len({word: None for word in text.split()})))
scenes = text.split('\n\n')
print('Number of scenes: {}'.format(len(scenes)))
sentence_count_scene = [scene.count('\n') for scene in scenes]
print('Average number of sentences in each scene: {}'.format(np.average(sentence_count_scene)))
sentences = [sentence for scene in scenes for sentence in scene.split('\n')]
print('Number of lines: {}'.format(len(sentences)))
word_count_sentence = [len(sentence.split()) for sentence in sentences]
print('Average number of words in each line: {}'.format(np.average(word_count_sentence)))
print()
print('The sentences {} to {}:'.format(*view_sentence_range))
print('\n'.join(text.split('\n')[view_sentence_range[0]:view_sentence_range[1]]))
###Output
_____no_output_____
###Markdown
Implement Preprocessing FunctionsThe first thing to do to any dataset is preprocessing. Implement the following preprocessing functions below:- Lookup Table- Tokenize Punctuation Lookup TableTo create a word embedding, you first need to transform the words to ids. In this function, create two dictionaries:- Dictionary to go from the words to an id, we'll call `vocab_to_int`- Dictionary to go from the id to word, we'll call `int_to_vocab`Return these dictionaries in the following tuple `(vocab_to_int, int_to_vocab)`
###Code
import numpy as np
import problem_unittests as tests
from collections import Counter
def create_lookup_tables(text):
"""
Create lookup tables for vocabulary
:param text: The text of tv scripts split into words
:return: A tuple of dicts (vocab_to_int, int_to_vocab)
"""
# TODO: Implement Function
# adapted from deep-learning/embeddings/utils.py
# sorts text most to least frequent
# keys are shifted to start at "1"
word_counts = Counter(text)
sorted_vocab = sorted(word_counts, key = word_counts.get, reverse = True)
int_to_vocab = {(j+1): word for j, word in enumerate(sorted_vocab)}
vocab_to_int = {word: j for j, word in int_to_vocab.items()}
return vocab_to_int, int_to_vocab
"""
DON'T MODIFY ANYTHING IN THIS CELL THAT IS BELOW THIS LINE
"""
tests.test_create_lookup_tables(create_lookup_tables)
###Output
_____no_output_____
###Markdown
Tokenize PunctuationWe'll be splitting the script into a word array using spaces as delimiters. However, punctuations like periods and exclamation marks make it hard for the neural network to distinguish between the word "bye" and "bye!".Implement the function `token_lookup` to return a dict that will be used to tokenize symbols like "!" into "||Exclamation_Mark||". Create a dictionary for the following symbols where the symbol is the key and value is the token:- Period ( . )- Comma ( , )- Quotation Mark ( " )- Semicolon ( ; )- Exclamation mark ( ! )- Question mark ( ? )- Left Parentheses ( ( )- Right Parentheses ( ) )- Dash ( -- )- Return ( \n )This dictionary will be used to token the symbols and add the delimiter (space) around it. This separates the symbols as it's own word, making it easier for the neural network to predict on the next word. Make sure you don't use a token that could be confused as a word. Instead of using the token "dash", try using something like "||dash||".
###Code
def token_lookup():
"""
Generate a dict to turn punctuation into a token.
:return: Tokenize dictionary where the key is the punctuation and the value is the token
"""
# TODO: Implement Function
# adapted from deep-learning/embeddings/utils.py
# [':', '||colon||']
symbols = [
['.', '||period||'],
[',', '||comma||'],
['"', '||quot_mark||'],
[';', '||semicolon||'],
['!', '||exclamation_mark||'],
['?', '||question_mark||'],
['(', '||L_paren||'],
[')', '||R_paren||'],
['--', '||hyphens||'],
['\n', '||newline||']
]
dict_tokens = dict([(s[0], s[1]) for s in symbols])
return dict_tokens
"""tests
DON'T MODIFY ANYTHING IN THIS CELL THAT IS BELOW THIS LINE
"""
tests.test_tokenize(token_lookup)
###Output
_____no_output_____
###Markdown
Preprocess all the data and save itRunning the code cell below will preprocess all the data and save it to file.
###Code
"""
DON'T MODIFY ANYTHING IN THIS CELL
"""
# Preprocess Training, Validation, and Testing Data
helper.preprocess_and_save_data(data_dir, token_lookup, create_lookup_tables)
###Output
_____no_output_____
###Markdown
Check PointThis is your first checkpoint. If you ever decide to come back to this notebook or have to restart the notebook, you can start from here. The preprocessed data has been saved to disk.
###Code
"""
DON'T MODIFY ANYTHING IN THIS CELL
"""
import helper
import numpy as np
import problem_unittests as tests
int_text, vocab_to_int, int_to_vocab, token_dict = helper.load_preprocess()
###Output
_____no_output_____
###Markdown
Build the Neural NetworkYou'll build the components necessary to build a RNN by implementing the following functions below:- get_inputs- get_init_cell- get_embed- build_rnn- build_nn- get_batches Check the Version of TensorFlow and Access to GPU
###Code
"""
DON'T MODIFY ANYTHING IN THIS CELL
"""
from distutils.version import LooseVersion
import warnings
import tensorflow as tf
# Check TensorFlow Version
assert LooseVersion(tf.__version__) >= LooseVersion('1.0'), 'Please use TensorFlow version 1.0 or newer'
print('TensorFlow Version: {}'.format(tf.__version__))
# Check for a GPU
if not tf.test.gpu_device_name():
warnings.warn('No GPU found. Please use a GPU to train your neural network.')
else:
print('Default GPU Device: {}'.format(tf.test.gpu_device_name()))
###Output
_____no_output_____
###Markdown
InputImplement the `get_inputs()` function to create TF Placeholders for the Neural Network. It should create the following placeholders:- Input text placeholder named "input" using the [TF Placeholder](https://www.tensorflow.org/api_docs/python/tf/placeholder) `name` parameter.- Targets placeholder- Learning Rate placeholderReturn the placeholders in the following tuple `(Input, Targets, LearningRate)`
###Code
def get_inputs():
"""
Create TF Placeholders for input, targets, and learning rate.
:return: Tuple (input, targets, learning rate)
"""
# TODO: Implement Function
inputs = tf.placeholder(tf.int32, [None, None], name = 'input')
targets = tf.placeholder(tf.int32, [None, None], name = 'targets')
learning_rate = tf.placeholder(tf.float32, name = 'learning_rate')
return (inputs, targets, learning_rate)
"""
DON'T MODIFY ANYTHING IN THIS CELL THAT IS BELOW THIS LINE
"""
tests.test_get_inputs(get_inputs)
###Output
_____no_output_____
###Markdown
Build RNN Cell and InitializeStack one or more [`BasicLSTMCells`](https://www.tensorflow.org/api_docs/python/tf/contrib/rnn/BasicLSTMCell) in a [`MultiRNNCell`](https://www.tensorflow.org/api_docs/python/tf/contrib/rnn/MultiRNNCell).- The Rnn size should be set using `rnn_size`- Initalize Cell State using the MultiRNNCell's [`zero_state()`](https://www.tensorflow.org/api_docs/python/tf/contrib/rnn/MultiRNNCellzero_state) function - Apply the name "initial_state" to the initial state using [`tf.identity()`](https://www.tensorflow.org/api_docs/python/tf/identity)Return the cell and initial state in the following tuple `(Cell, InitialState)`
###Code
def get_init_cell(batch_size, rnn_size):
"""
Create an RNN Cell and initialize it.
:param batch_size: Size of batches
:param rnn_size: Size of RNNs (int)
:return: Tuple (cell, initialize state)
"""
# TODO: Implement Function
def build_cell(rnn_size):
lstm = tf.contrib.rnn.BasicLSTMCell(rnn_size)
# drop = tf.contrib.rnn.DropoutWrapper(lstm, output_keep_prob = 0.8)
return lstm
Cell = tf.contrib.rnn.MultiRNNCell([build_cell(rnn_size) for _ in range(2)])
Init = Cell.zero_state(batch_size, tf.float32)
InitialState = tf.identity(Init, name = 'initial_state')
return (Cell, InitialState)
"""
DON'T MODIFY ANYTHING IN THIS CELL THAT IS BELOW THIS LINE
"""
tests.test_get_init_cell(get_init_cell)
###Output
_____no_output_____
###Markdown
Word EmbeddingApply embedding to `input_data` using TensorFlow. Return the embedded sequence.
###Code
def get_embed(input_data, vocab_size, embed_dim):
"""
Create embedding for <input_data>.
:param input_data: TF placeholder for text input.
:param vocab_size: Number of words in vocabulary.
:param embed_dim: Number of embedding dimensions
:return: Embedded input.
"""
# TODO: Implement Function
embedding = tf.Variable(tf.random_uniform((vocab_size, embed_dim), -1, 1))
embed = tf.nn.embedding_lookup(embedding, input_data)
return embed
"""
DON'T MODIFY ANYTHING IN THIS CELL THAT IS BELOW THIS LINE
"""
tests.test_get_embed(get_embed)
###Output
_____no_output_____
###Markdown
Build RNNYou created a RNN Cell in the `get_init_cell()` function. Time to use the cell to create a RNN.- Build the RNN using the [`tf.nn.dynamic_rnn()`](https://www.tensorflow.org/api_docs/python/tf/nn/dynamic_rnn) - Apply the name "final_state" to the final state using [`tf.identity()`](https://www.tensorflow.org/api_docs/python/tf/identity)Return the outputs and final_state state in the following tuple `(Outputs, FinalState)`
###Code
def build_rnn(cell, inputs):
"""
Create a RNN using a RNN Cell
:param cell: RNN Cell
:param inputs: Input text data
:return: Tuple (Outputs, Final State)
"""
# TODO: Implement Function
Outputs, FinalState = tf.nn.dynamic_rnn(cell, inputs, dtype = tf.float32)
FinalState = tf.identity(FinalState, name = 'final_state')
return Outputs, FinalState
"""
DON'T MODIFY ANYTHING IN THIS CELL THAT IS BELOW THIS LINE
"""
tests.test_build_rnn(build_rnn)
###Output
_____no_output_____
###Markdown
Build the Neural NetworkApply the functions you implemented above to:- Apply embedding to `input_data` using your `get_embed(input_data, vocab_size, embed_dim)` function.- Build RNN using `cell` and your `build_rnn(cell, inputs)` function.- Apply a fully connected layer with a linear activation and `vocab_size` as the number of outputs.Return the logits and final state in the following tuple (Logits, FinalState)
###Code
def build_nn(cell, rnn_size, input_data, vocab_size, embed_dim):
"""
Build part of the neural network
:param cell: RNN cell
:param rnn_size: Size of rnns
:param input_data: Input data
:param vocab_size: Vocabulary size
:param embed_dim: Number of embedding dimensions
:return: Tuple (Logits, FinalState)
"""
# TODO: Implement Function
# get embed return: Embedded input
embed = get_embed(input_data, vocab_size, embed_dim)
# build_rnn returns: Outputs, FinalState
RNN_Outputs, FinalState = build_rnn(cell, embed)
# apply FC layer
Logits = tf.contrib.layers.fully_connected(RNN_Outputs, vocab_size, activation_fn = None,\
weights_initializer = tf.truncated_normal_initializer(stddev = 0.1),\
biases_initializer = tf.zeros_initializer())
return Logits, FinalState
"""
DON'T MODIFY ANYTHING IN THIS CELL THAT IS BELOW THIS LINE
"""
tests.test_build_nn(build_nn)
###Output
_____no_output_____
###Markdown
BatchesImplement `get_batches` to create batches of input and targets using `int_text`. The batches should be a Numpy array with the shape `(number of batches, 2, batch size, sequence length)`. Each batch contains two elements:- The first element is a single batch of **input** with the shape `[batch size, sequence length]`- The second element is a single batch of **targets** with the shape `[batch size, sequence length]`If you can't fill the last batch with enough data, drop the last batch.For exmple, `get_batches([1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20], 3, 2)` would return a Numpy array of the following:```[ First Batch [ Batch of Input [[ 1 2], [ 7 8], [13 14]] Batch of targets [[ 2 3], [ 8 9], [14 15]] ] Second Batch [ Batch of Input [[ 3 4], [ 9 10], [15 16]] Batch of targets [[ 4 5], [10 11], [16 17]] ] Third Batch [ Batch of Input [[ 5 6], [11 12], [17 18]] Batch of targets [[ 6 7], [12 13], [18 1]] ]]```Notice that the last target value in the last batch is the first input value of the first batch. In this case, `1`. This is a common technique used when creating sequence batches, although it is rather unintuitive.
###Code
import numpy as np
def get_batches(int_text, batch_size, seq_length):
"""
Return batches of input and target
:param int_text: Text with the words replaced by their ids : array shape = (1,)
:param batch_size: The size of batch : int
:param seq_length: The length of sequence : int
:return: Batches as a Numpy array with the shape (number of batches, 2, batch size, sequence length)
"""
# find num_batches
batch_len = batch_size*seq_length
num_batches = len(int_text)//batch_len
count_len = seq_length*num_batches
batch_range = num_batches*batch_len
# initialize B
B = np.zeros((num_batches, 2, batch_size, seq_length), np.int)
for i in range(num_batches):
for j in range(batch_size):
for k in range(seq_length):
# inputs
B[i,0,j,k] = np.add(B[i,0,j,k], int_text[(k+(j*count_len)+(i*seq_length))])
#targets
B[i,1,j,k] = np.add(B[i,1,j,k], int_text[(k+(j*count_len)+(i*seq_length)+1)%batch_range])
return B
"""
DON'T MODIFY ANYTHING IN THIS CELL THAT IS BELOW THIS LINE
"""
tests.test_get_batches(get_batches)
###Output
_____no_output_____
###Markdown
Neural Network Training HyperparametersTune the following parameters:- Set `num_epochs` to the number of epochs.- Set `batch_size` to the batch size.- Set `rnn_size` to the size of the RNNs.- Set `embed_dim` to the size of the embedding.- Set `seq_length` to the length of sequence.- Set `learning_rate` to the learning rate.- Set `show_every_n_batches` to the number of batches the neural network should print progress.
###Code
# Number of Epochs
num_epochs = 100
# Batch Size
batch_size = 128
# RNN Size
rnn_size = 256
# Embedding Dimension Size
embed_dim = 128
# Sequence Length
seq_length = 12
# Learning Rate
learning_rate = 0.01
# Show stats for every n number of batches
show_every_n_batches = 100
"""
DON'T MODIFY ANYTHING IN THIS CELL THAT IS BELOW THIS LINE
"""
save_dir = './save'
###Output
_____no_output_____
###Markdown
Build the GraphBuild the graph using the neural network you implemented.
###Code
"""
DON'T MODIFY ANYTHING IN THIS CELL
"""
from tensorflow.contrib import seq2seq
train_graph = tf.Graph()
with train_graph.as_default():
vocab_size = len(int_to_vocab)
input_text, targets, lr = get_inputs()
input_data_shape = tf.shape(input_text)
cell, initial_state = get_init_cell(input_data_shape[0], rnn_size)
logits, final_state = build_nn(cell, rnn_size, input_text, vocab_size, embed_dim)
# Probabilities for generating words
probs = tf.nn.softmax(logits, name='probs')
# Loss function
cost = seq2seq.sequence_loss(
logits,
targets,
tf.ones([input_data_shape[0], input_data_shape[1]]))
# Optimizer
optimizer = tf.train.AdamOptimizer(lr)
# Gradient Clipping
gradients = optimizer.compute_gradients(cost)
capped_gradients = [(tf.clip_by_value(grad, -1., 1.), var) for grad, var in gradients if grad is not None]
train_op = optimizer.apply_gradients(capped_gradients)
###Output
_____no_output_____
###Markdown
TrainTrain the neural network on the preprocessed data. If you have a hard time getting a good loss, check the [forums](https://discussions.udacity.com/) to see if anyone is having the same problem.
###Code
"""
DON'T MODIFY ANYTHING IN THIS CELL
"""
batches = get_batches(int_text, batch_size, seq_length)
with tf.Session(graph=train_graph) as sess:
sess.run(tf.global_variables_initializer())
for epoch_i in range(num_epochs):
state = sess.run(initial_state, {input_text: batches[0][0]})
for batch_i, (x, y) in enumerate(batches):
feed = {
input_text: x,
targets: y,
initial_state: state,
lr: learning_rate}
train_loss, state, _ = sess.run([cost, final_state, train_op], feed)
# Show every <show_every_n_batches> batches
if (epoch_i * len(batches) + batch_i) % show_every_n_batches == 0:
print('Epoch {:>3} Batch {:>4}/{} train_loss = {:.3f}'.format(
epoch_i,
batch_i,
len(batches),
train_loss))
# Save Model
saver = tf.train.Saver()
saver.save(sess, save_dir)
print('Model Trained and Saved')
###Output
_____no_output_____
###Markdown
Save ParametersSave `seq_length` and `save_dir` for generating a new TV script.
###Code
"""
DON'T MODIFY ANYTHING IN THIS CELL
"""
# Save parameters for checkpoint
helper.save_params((seq_length, save_dir))
###Output
_____no_output_____
###Markdown
Checkpoint
###Code
"""
DON'T MODIFY ANYTHING IN THIS CELL
"""
import tensorflow as tf
# import numpy as np
# imported with get_batches cell
import helper
import problem_unittests as tests
_, vocab_to_int, int_to_vocab, token_dict = helper.load_preprocess()
seq_length, load_dir = helper.load_params()
###Output
_____no_output_____
###Markdown
Implement Generate Functions Get TensorsGet tensors from `loaded_graph` using the function [`get_tensor_by_name()`](https://www.tensorflow.org/api_docs/python/tf/Graphget_tensor_by_name). Get the tensors using the following names:- "input:0"- "initial_state:0"- "final_state:0"- "probs:0"Return the tensors in the following tuple `(InputTensor, InitialStateTensor, FinalStateTensor, ProbsTensor)`
###Code
def get_tensors(loaded_graph):
"""
Get input, initial state, final state, and probabilities tensor from <loaded_graph>
:param loaded_graph: TensorFlow graph loaded from file
:return: Tuple (InputTensor, InitialStateTensor, FinalStateTensor, ProbsTensor)
"""
# TODO: Implement Function
InputTensor = loaded_graph.get_tensor_by_name("input:0")
InitialStateTensor = loaded_graph.get_tensor_by_name("initial_state:0")
FinalStateTensor = loaded_graph.get_tensor_by_name("final_state:0")
ProbsTensor = loaded_graph.get_tensor_by_name("probs:0")
return (InputTensor, InitialStateTensor, FinalStateTensor, ProbsTensor)
"""
DON'T MODIFY ANYTHING IN THIS CELL THAT IS BELOW THIS LINE
"""
tests.test_get_tensors(get_tensors)
###Output
_____no_output_____
###Markdown
Choose WordImplement the `pick_word()` function to select the next word using `probabilities`.
###Code
def pick_word(probabilities, int_to_vocab):
"""
Pick the next word in the generated text
:param probabilities: Probabilites of the next word : array
:param int_to_vocab: Dictionary of word ids as the keys and words as the values
:return: String of the predicted word
"""
# TODO: Implement Function
probs = np.array(probabilities)
labels = probs.argsort()[-4:][::-1]
rand_label = np.random.choice(labels, 1, p = [0.5, 0.25, 0.15, 0.1])
word = int_to_vocab[rand_label[0]]
return word
"""
DON'T MODIFY ANYTHING IN THIS CELL THAT IS BELOW THIS LINE
"""
tests.test_pick_word(pick_word)
###Output
_____no_output_____
###Markdown
Generate TV ScriptThis will generate the TV script for you. Set `gen_length` to the length of TV script you want to generate.
###Code
gen_length = 300
# homer_simpson, moe_szyslak, or Barney_Gumble
prime_word = 'moe_szyslak'
"""
DON'T MODIFY ANYTHING IN THIS CELL THAT IS BELOW THIS LINE
"""
loaded_graph = tf.Graph()
with tf.Session(graph=loaded_graph) as sess:
# Load saved model
loader = tf.train.import_meta_graph(load_dir + '.meta')
loader.restore(sess, load_dir)
# Get Tensors from loaded model
input_text, initial_state, final_state, probs = get_tensors(loaded_graph)
# Sentences generation setup
gen_sentences = [prime_word + ':']
prev_state = sess.run(initial_state, {input_text: np.array([[1]])})
# Generate sentences
for n in range(gen_length):
# Dynamic Input
dyn_input = [[vocab_to_int[word] for word in gen_sentences[-seq_length:]]]
dyn_seq_length = len(dyn_input[0])
# Get Prediction
probabilities, prev_state = sess.run(
[probs, final_state],
{input_text: dyn_input, initial_state: prev_state})
pred_word = pick_word(probabilities[dyn_seq_length-1], int_to_vocab)
gen_sentences.append(pred_word)
# Remove tokens
tv_script = ' '.join(gen_sentences)
for key, token in token_dict.items():
ending = ' ' if key in ['\n', '(', '"'] else ''
tv_script = tv_script.replace(' ' + token.lower(), key)
tv_script = tv_script.replace('\n ', '\n')
tv_script = tv_script.replace('( ', '(')
print(tv_script)
###Output
_____no_output_____ |
src/data/RNAseq_RTG_expression/get_RTG-Expression-Table_zero-removed.ipynb | ###Markdown
Analysis RTG gene expression Reference- Single-cell RNA-seq reveals intrinsic and extrinsic regulatory heterogeneity in yeast responding to stress - Article. https://doi.org/10.1371/journal.pbio.2004050 - RNA sequencing data(GSA102475). https://www.ncbi.nlm.nih.gov/geo/query/acc.cgi?acc=GSE102475
###Code
# Retrieve table
import pandas as pd
import numbers
df = pd.read_table("data/GSE102475_GASCH_NaCl-scRNAseq_NormData.txt")
def get_key(df, value):
return [k for k,v in df.items() if value==v]
###Output
_____no_output_____
###Markdown
Check availibility of RTG Genes
###Code
# Gene names
alias = {
"RTG1": "YOL067C",
"RTG2": "YGL252C",
"RTG3": "YBL103C",
"BMH1": "YER177W",
"MKS1": "YNL076W",
"CIT2": "YCR005C"
}
for k in alias.keys():
if alias[k] in list(df["gene"]):
print("{} is measured".format(k))
else:
print("{} not found".format(k))
###Output
RTG1 is measured
RTG2 is measured
RTG3 is measured
BMH1 is measured
MKS1 is measured
CIT2 is measured
###Markdown
Get RTG gene expression table
###Code
df_filt = df[df["gene"].isin(alias.values())]
# filtered zeros
df_filt_zeroRemoved = df_filt
for key in df_filt.keys():
col = df_filt[key]
if 0.00 in list(col):
del df_filt_zeroRemoved[key]
# a to columndd alias
alias_ns = [ get_key(alias, ali)[0] for ali in df_filt_zeroRemoved["gene"]]
df_filt_zeroRemoved["gene_alias"] = alias_ns
df_filt_zeroRemoved
df_filt_zeroRemoved.to_csv("data/RTG-Expression-Table_GSE102475.csv", index=False)
###Output
_____no_output_____ |
data_collection_webscraping.ipynb | ###Markdown
Space X Landing Prediction Web scraping Falcon 9 Lauch RecordsWeb scraping to collect Falcon 9 historical launch records from a *Wikipedia* page titled List of Falcon 9 and Falcon Heavy launches.Target Url: https://en.wikipedia.org/wiki/List_of_Falcon_9_and_Falcon_Heavy_launches ObjectivesWeb scrap Falcon 9 launch records with BeautifulSoup:- Extract a Falcon 9 launch records HTML table from Wikipedia- Parse the table and convert it into a Pandas data frame Importing Libraries
###Code
import sys
import requests
import pandas as pd
from bs4 import BeautifulSoup
import re
import unicodedata
###Output
_____no_output_____
###Markdown
Assigning Static URL
###Code
static_url = "https://en.wikipedia.org/w/index.php?title=List_of_Falcon_9_and_Falcon_Heavy_launches&oldid=1027686922"
###Output
_____no_output_____
###Markdown
Calling Helper Functions for Scrapping HTML table.
###Code
# This function returns the data and time from the HTML table cell
def date_time(table_cells):
return[data_time.strip() for data_time in list(table_cells.strings)][0:2]
# This function returns the booster version from the HTML table cell
def booster_version(table_cells):
out = ''.join([booster_version for i,booster_version in enumerate(table_cells.strings) if i%2 == 0][0:-1])
return out
# This function returns the landing status from the HTML table cell
def landing_status(table_cells):
out = [i for i in table_cells.strings][0]
return out
# This function returns the mass from the HTML table cell
def get_mass(table_cells):
mass=unicodedata.normalize("NFKD", table_cells.text).strip()
if mass:
mass.find("kg")
new_mass = mass[0:mass.find("kg")+2]
else:
new_mass = 0
return new_mass
# This function returns the columns from the HTML table cell
def extract_column_from_header(row):
if (row.br):
row.br.extract()
if row.a:
row.a.extract()
if row.sup:
row.sup.extract()
column_name = ' '.join(row.contents)
# Filter the digit and empty names
if not(column_name.strip().isdigit()):
column_name = column_name.strip()
return column_name
###Output
_____no_output_____
###Markdown
Request the Falcon9 Launch Wiki page from its URL.
###Code
response = requests.get(static_url)
html_doc = response.text
###Output
_____no_output_____
###Markdown
Using BeautifulSoup to create a object from response text content
###Code
soup = BeautifulSoup(html_doc, 'html.parser')
print(soup.title)
###Output
<title>List of Falcon 9 and Falcon Heavy launches - Wikipedia</title>
###Markdown
Extract all column/variable names from the HTML table header
###Code
html_tables = soup.find_all('table')
first_launch_table = html_tables[2]
print(first_launch_table)
###Output
<table class="wikitable plainrowheaders collapsible" style="width: 100%;">
<tbody><tr>
<th scope="col">Flight No.
</th>
<th scope="col">Date and<br/>time (<a href="/wiki/Coordinated_Universal_Time" title="Coordinated Universal Time">UTC</a>)
</th>
<th scope="col"><a href="/wiki/List_of_Falcon_9_first-stage_boosters" title="List of Falcon 9 first-stage boosters">Version,<br/>Booster</a> <sup class="reference" id="cite_ref-booster_11-0"><a href="#cite_note-booster-11">[b]</a></sup>
</th>
<th scope="col">Launch site
</th>
<th scope="col">Payload<sup class="reference" id="cite_ref-Dragon_12-0"><a href="#cite_note-Dragon-12">[c]</a></sup>
</th>
<th scope="col">Payload mass
</th>
<th scope="col">Orbit
</th>
<th scope="col">Customer
</th>
<th scope="col">Launch<br/>outcome
</th>
<th scope="col"><a href="/wiki/Falcon_9_first-stage_landing_tests" title="Falcon 9 first-stage landing tests">Booster<br/>landing</a>
</th></tr>
<tr>
<th rowspan="2" scope="row" style="text-align:center;">1
</th>
<td>4 June 2010,<br/>18:45
</td>
<td><a href="/wiki/Falcon_9_v1.0" title="Falcon 9 v1.0">F9 v1.0</a><sup class="reference" id="cite_ref-MuskMay2012_13-0"><a href="#cite_note-MuskMay2012-13">[7]</a></sup><br/>B0003.1<sup class="reference" id="cite_ref-block_numbers_14-0"><a href="#cite_note-block_numbers-14">[8]</a></sup>
</td>
<td><a href="/wiki/Cape_Canaveral_Space_Force_Station" title="Cape Canaveral Space Force Station">CCAFS</a>,<br/><a href="/wiki/Cape_Canaveral_Space_Launch_Complex_40" title="Cape Canaveral Space Launch Complex 40">SLC-40</a>
</td>
<td><a href="/wiki/Dragon_Spacecraft_Qualification_Unit" title="Dragon Spacecraft Qualification Unit">Dragon Spacecraft Qualification Unit</a>
</td>
<td>
</td>
<td><a href="/wiki/Low_Earth_orbit" title="Low Earth orbit">LEO</a>
</td>
<td><a href="/wiki/SpaceX" title="SpaceX">SpaceX</a>
</td>
<td class="table-success" style="background: LightGreen; color: black; vertical-align: middle; text-align: center;">Success
</td>
<td class="table-failure" style="background: #ffbbbb; color: black; vertical-align: middle; text-align: center;">Failure<sup class="reference" id="cite_ref-ns20110930_15-0"><a href="#cite_note-ns20110930-15">[9]</a></sup><sup class="reference" id="cite_ref-16"><a href="#cite_note-16">[10]</a></sup><br/><small>(parachute)</small>
</td></tr>
<tr>
<td colspan="9">First flight of Falcon 9 v1.0.<sup class="reference" id="cite_ref-sfn20100604_17-0"><a href="#cite_note-sfn20100604-17">[11]</a></sup> Used a boilerplate version of Dragon capsule which was not designed to separate from the second stage.<small>(<a href="#First_flight_of_Falcon_9">more details below</a>)</small> Attempted to recover the first stage by parachuting it into the ocean, but it burned up on reentry, before the parachutes even deployed.<sup class="reference" id="cite_ref-parachute_18-0"><a href="#cite_note-parachute-18">[12]</a></sup>
</td></tr>
<tr>
<th rowspan="2" scope="row" style="text-align:center;">2
</th>
<td>8 December 2010,<br/>15:43<sup class="reference" id="cite_ref-spaceflightnow_Clark_Launch_Report_19-0"><a href="#cite_note-spaceflightnow_Clark_Launch_Report-19">[13]</a></sup>
</td>
<td><a href="/wiki/Falcon_9_v1.0" title="Falcon 9 v1.0">F9 v1.0</a><sup class="reference" id="cite_ref-MuskMay2012_13-1"><a href="#cite_note-MuskMay2012-13">[7]</a></sup><br/>B0004.1<sup class="reference" id="cite_ref-block_numbers_14-1"><a href="#cite_note-block_numbers-14">[8]</a></sup>
</td>
<td><a href="/wiki/Cape_Canaveral_Space_Force_Station" title="Cape Canaveral Space Force Station">CCAFS</a>,<br/><a href="/wiki/Cape_Canaveral_Space_Launch_Complex_40" title="Cape Canaveral Space Launch Complex 40">SLC-40</a>
</td>
<td><a href="/wiki/SpaceX_Dragon" title="SpaceX Dragon">Dragon</a> <a class="mw-redirect" href="/wiki/COTS_Demo_Flight_1" title="COTS Demo Flight 1">demo flight C1</a><br/>(Dragon C101)
</td>
<td>
</td>
<td><a href="/wiki/Low_Earth_orbit" title="Low Earth orbit">LEO</a> (<a href="/wiki/International_Space_Station" title="International Space Station">ISS</a>)
</td>
<td><div class="plainlist">
<ul><li><a href="/wiki/NASA" title="NASA">NASA</a> (<a href="/wiki/Commercial_Orbital_Transportation_Services" title="Commercial Orbital Transportation Services">COTS</a>)</li>
<li><a href="/wiki/National_Reconnaissance_Office" title="National Reconnaissance Office">NRO</a></li></ul>
</div>
</td>
<td class="table-success" style="background: LightGreen; color: black; vertical-align: middle; text-align: center;">Success<sup class="reference" id="cite_ref-ns20110930_15-1"><a href="#cite_note-ns20110930-15">[9]</a></sup>
</td>
<td class="table-failure" style="background: #ffbbbb; color: black; vertical-align: middle; text-align: center;">Failure<sup class="reference" id="cite_ref-ns20110930_15-2"><a href="#cite_note-ns20110930-15">[9]</a></sup><sup class="reference" id="cite_ref-20"><a href="#cite_note-20">[14]</a></sup><br/><small>(parachute)</small>
</td></tr>
<tr>
<td colspan="9">Maiden flight of <a class="mw-redirect" href="/wiki/Dragon_capsule" title="Dragon capsule">Dragon capsule</a>, consisting of over 3 hours of testing thruster maneuvering and reentry.<sup class="reference" id="cite_ref-spaceflightnow_Clark_unleashing_Dragon_21-0"><a href="#cite_note-spaceflightnow_Clark_unleashing_Dragon-21">[15]</a></sup> Attempted to recover the first stage by parachuting it into the ocean, but it disintegrated upon reentry, before the parachutes were deployed.<sup class="reference" id="cite_ref-parachute_18-1"><a href="#cite_note-parachute-18">[12]</a></sup> <small>(<a href="#COTS_demo_missions">more details below</a>)</small> It also included two <a href="/wiki/CubeSat" title="CubeSat">CubeSats</a>,<sup class="reference" id="cite_ref-NRO_Taps_Boeing_for_Next_Batch_of_CubeSats_22-0"><a href="#cite_note-NRO_Taps_Boeing_for_Next_Batch_of_CubeSats-22">[16]</a></sup> and a wheel of <a href="/wiki/Brou%C3%A8re" title="Brouère">Brouère</a> cheese.
</td></tr>
<tr>
<th rowspan="2" scope="row" style="text-align:center;">3
</th>
<td>22 May 2012,<br/>07:44<sup class="reference" id="cite_ref-BBC_new_era_23-0"><a href="#cite_note-BBC_new_era-23">[17]</a></sup>
</td>
<td><a href="/wiki/Falcon_9_v1.0" title="Falcon 9 v1.0">F9 v1.0</a><sup class="reference" id="cite_ref-MuskMay2012_13-2"><a href="#cite_note-MuskMay2012-13">[7]</a></sup><br/>B0005.1<sup class="reference" id="cite_ref-block_numbers_14-2"><a href="#cite_note-block_numbers-14">[8]</a></sup>
</td>
<td><a href="/wiki/Cape_Canaveral_Space_Force_Station" title="Cape Canaveral Space Force Station">CCAFS</a>,<br/><a href="/wiki/Cape_Canaveral_Space_Launch_Complex_40" title="Cape Canaveral Space Launch Complex 40">SLC-40</a>
</td>
<td><a href="/wiki/SpaceX_Dragon" title="SpaceX Dragon">Dragon</a> <a class="mw-redirect" href="/wiki/Dragon_C2%2B" title="Dragon C2+">demo flight C2+</a><sup class="reference" id="cite_ref-C2_24-0"><a href="#cite_note-C2-24">[18]</a></sup><br/>(Dragon C102)
</td>
<td>525 kg (1,157 lb)<sup class="reference" id="cite_ref-25"><a href="#cite_note-25">[19]</a></sup>
</td>
<td><a href="/wiki/Low_Earth_orbit" title="Low Earth orbit">LEO</a> (<a href="/wiki/International_Space_Station" title="International Space Station">ISS</a>)
</td>
<td><a href="/wiki/NASA" title="NASA">NASA</a> (<a href="/wiki/Commercial_Orbital_Transportation_Services" title="Commercial Orbital Transportation Services">COTS</a>)
</td>
<td class="table-success" style="background: LightGreen; color: black; vertical-align: middle; text-align: center;">Success<sup class="reference" id="cite_ref-26"><a href="#cite_note-26">[20]</a></sup>
</td>
<td class="table-noAttempt" style="background: #ececec; color: black; vertical-align: middle; white-space: nowrap; text-align: center;">No attempt
</td></tr>
<tr>
<td colspan="9">Dragon spacecraft demonstrated a series of tests before it was allowed to approach the <a href="/wiki/International_Space_Station" title="International Space Station">International Space Station</a>. Two days later, it became the first commercial spacecraft to board the ISS.<sup class="reference" id="cite_ref-BBC_new_era_23-1"><a href="#cite_note-BBC_new_era-23">[17]</a></sup> <small>(<a href="#COTS_demo_missions">more details below</a>)</small>
</td></tr>
<tr>
<th rowspan="3" scope="row" style="text-align:center;">4
</th>
<td rowspan="2">8 October 2012,<br/>00:35<sup class="reference" id="cite_ref-SFN_LLog_27-0"><a href="#cite_note-SFN_LLog-27">[21]</a></sup>
</td>
<td rowspan="2"><a href="/wiki/Falcon_9_v1.0" title="Falcon 9 v1.0">F9 v1.0</a><sup class="reference" id="cite_ref-MuskMay2012_13-3"><a href="#cite_note-MuskMay2012-13">[7]</a></sup><br/>B0006.1<sup class="reference" id="cite_ref-block_numbers_14-3"><a href="#cite_note-block_numbers-14">[8]</a></sup>
</td>
<td rowspan="2"><a href="/wiki/Cape_Canaveral_Space_Force_Station" title="Cape Canaveral Space Force Station">CCAFS</a>,<br/><a href="/wiki/Cape_Canaveral_Space_Launch_Complex_40" title="Cape Canaveral Space Launch Complex 40">SLC-40</a>
</td>
<td><a href="/wiki/SpaceX_CRS-1" title="SpaceX CRS-1">SpaceX CRS-1</a><sup class="reference" id="cite_ref-sxManifest20120925_28-0"><a href="#cite_note-sxManifest20120925-28">[22]</a></sup><br/>(Dragon C103)
</td>
<td>4,700 kg (10,400 lb)
</td>
<td><a href="/wiki/Low_Earth_orbit" title="Low Earth orbit">LEO</a> (<a href="/wiki/International_Space_Station" title="International Space Station">ISS</a>)
</td>
<td><a href="/wiki/NASA" title="NASA">NASA</a> (<a href="/wiki/Commercial_Resupply_Services" title="Commercial Resupply Services">CRS</a>)
</td>
<td class="table-success" style="background: LightGreen; color: black; vertical-align: middle; text-align: center;">Success
</td>
<td rowspan="2" style="background:#ececec; text-align:center;"><span class="nowrap">No attempt</span>
</td></tr>
<tr>
<td><a href="/wiki/Orbcomm_(satellite)" title="Orbcomm (satellite)">Orbcomm-OG2</a><sup class="reference" id="cite_ref-Orbcomm_29-0"><a href="#cite_note-Orbcomm-29">[23]</a></sup>
</td>
<td>172 kg (379 lb)<sup class="reference" id="cite_ref-gunter-og2_30-0"><a href="#cite_note-gunter-og2-30">[24]</a></sup>
</td>
<td><a href="/wiki/Low_Earth_orbit" title="Low Earth orbit">LEO</a>
</td>
<td><a href="/wiki/Orbcomm" title="Orbcomm">Orbcomm</a>
</td>
<td class="table-partial" style="background: wheat; color: black; vertical-align: middle; text-align: center;">Partial failure<sup class="reference" id="cite_ref-nyt-20121030_31-0"><a href="#cite_note-nyt-20121030-31">[25]</a></sup>
</td></tr>
<tr>
<td colspan="9">CRS-1 was successful, but the <a href="/wiki/Secondary_payload" title="Secondary payload">secondary payload</a> was inserted into an abnormally low orbit and subsequently lost. This was due to one of the nine <a href="/wiki/SpaceX_Merlin" title="SpaceX Merlin">Merlin engines</a> shutting down during the launch, and NASA declining a second reignition, as per <a href="/wiki/International_Space_Station" title="International Space Station">ISS</a> visiting vehicle safety rules, the primary payload owner is contractually allowed to decline a second reignition. NASA stated that this was because SpaceX could not guarantee a high enough likelihood of the second stage completing the second burn successfully which was required to avoid any risk of secondary payload's collision with the ISS.<sup class="reference" id="cite_ref-OrbcommTotalLoss_32-0"><a href="#cite_note-OrbcommTotalLoss-32">[26]</a></sup><sup class="reference" id="cite_ref-sn20121011_33-0"><a href="#cite_note-sn20121011-33">[27]</a></sup><sup class="reference" id="cite_ref-34"><a href="#cite_note-34">[28]</a></sup>
</td></tr>
<tr>
<th rowspan="2" scope="row" style="text-align:center;">5
</th>
<td>1 March 2013,<br/>15:10
</td>
<td><a href="/wiki/Falcon_9_v1.0" title="Falcon 9 v1.0">F9 v1.0</a><sup class="reference" id="cite_ref-MuskMay2012_13-4"><a href="#cite_note-MuskMay2012-13">[7]</a></sup><br/>B0007.1<sup class="reference" id="cite_ref-block_numbers_14-4"><a href="#cite_note-block_numbers-14">[8]</a></sup>
</td>
<td><a href="/wiki/Cape_Canaveral_Space_Force_Station" title="Cape Canaveral Space Force Station">CCAFS</a>,<br/><a href="/wiki/Cape_Canaveral_Space_Launch_Complex_40" title="Cape Canaveral Space Launch Complex 40">SLC-40</a>
</td>
<td><a href="/wiki/SpaceX_CRS-2" title="SpaceX CRS-2">SpaceX CRS-2</a><sup class="reference" id="cite_ref-sxManifest20120925_28-1"><a href="#cite_note-sxManifest20120925-28">[22]</a></sup><br/>(Dragon C104)
</td>
<td>4,877 kg (10,752 lb)
</td>
<td><a href="/wiki/Low_Earth_orbit" title="Low Earth orbit">LEO</a> (<a class="mw-redirect" href="/wiki/ISS" title="ISS">ISS</a>)
</td>
<td><a href="/wiki/NASA" title="NASA">NASA</a> (<a href="/wiki/Commercial_Resupply_Services" title="Commercial Resupply Services">CRS</a>)
</td>
<td class="table-success" style="background: LightGreen; color: black; vertical-align: middle; text-align: center;">Success
</td>
<td class="table-noAttempt" style="background: #ececec; color: black; vertical-align: middle; white-space: nowrap; text-align: center;">No attempt
</td></tr>
<tr>
<td colspan="9">Last launch of the original Falcon 9 v1.0 <a href="/wiki/Launch_vehicle" title="Launch vehicle">launch vehicle</a>, first use of the unpressurized trunk section of Dragon.<sup class="reference" id="cite_ref-sxf9_20110321_35-0"><a href="#cite_note-sxf9_20110321-35">[29]</a></sup>
</td></tr>
<tr>
<th rowspan="2" scope="row" style="text-align:center;">6
</th>
<td>29 September 2013,<br/>16:00<sup class="reference" id="cite_ref-pa20130930_36-0"><a href="#cite_note-pa20130930-36">[30]</a></sup>
</td>
<td><a href="/wiki/Falcon_9_v1.1" title="Falcon 9 v1.1">F9 v1.1</a><sup class="reference" id="cite_ref-MuskMay2012_13-5"><a href="#cite_note-MuskMay2012-13">[7]</a></sup><br/>B1003<sup class="reference" id="cite_ref-block_numbers_14-5"><a href="#cite_note-block_numbers-14">[8]</a></sup>
</td>
<td><a class="mw-redirect" href="/wiki/Vandenberg_Air_Force_Base" title="Vandenberg Air Force Base">VAFB</a>,<br/><a href="/wiki/Vandenberg_Space_Launch_Complex_4" title="Vandenberg Space Launch Complex 4">SLC-4E</a>
</td>
<td><a href="/wiki/CASSIOPE" title="CASSIOPE">CASSIOPE</a><sup class="reference" id="cite_ref-sxManifest20120925_28-2"><a href="#cite_note-sxManifest20120925-28">[22]</a></sup><sup class="reference" id="cite_ref-CASSIOPE_MDA_37-0"><a href="#cite_note-CASSIOPE_MDA-37">[31]</a></sup>
</td>
<td>500 kg (1,100 lb)
</td>
<td><a href="/wiki/Polar_orbit" title="Polar orbit">Polar orbit</a> <a href="/wiki/Low_Earth_orbit" title="Low Earth orbit">LEO</a>
</td>
<td><a href="/wiki/Maxar_Technologies" title="Maxar Technologies">MDA</a>
</td>
<td class="table-success" style="background: LightGreen; color: black; vertical-align: middle; text-align: center;">Success<sup class="reference" id="cite_ref-pa20130930_36-1"><a href="#cite_note-pa20130930-36">[30]</a></sup>
</td>
<td class="table-no2" style="background: #ffdddd; color: black; vertical-align: middle; text-align: center;">Uncontrolled<br/><small>(ocean)</small><sup class="reference" id="cite_ref-ocean_landing_38-0"><a href="#cite_note-ocean_landing-38">[d]</a></sup>
</td></tr>
<tr>
<td colspan="9">First commercial mission with a private customer, first launch from Vandenberg, and demonstration flight of Falcon 9 v1.1 with an improved 13-tonne to LEO capacity.<sup class="reference" id="cite_ref-sxf9_20110321_35-1"><a href="#cite_note-sxf9_20110321-35">[29]</a></sup> After separation from the second stage carrying Canadian commercial and scientific satellites, the first stage booster performed a controlled reentry,<sup class="reference" id="cite_ref-39"><a href="#cite_note-39">[32]</a></sup> and an <a href="/wiki/Falcon_9_first-stage_landing_tests" title="Falcon 9 first-stage landing tests">ocean touchdown test</a> for the first time. This provided good test data, even though the booster started rolling as it neared the ocean, leading to the shutdown of the central engine as the roll depleted it of fuel, resulting in a hard impact with the ocean.<sup class="reference" id="cite_ref-pa20130930_36-2"><a href="#cite_note-pa20130930-36">[30]</a></sup> This was the first known attempt of a rocket engine being lit to perform a supersonic retro propulsion, and allowed SpaceX to enter a public-private partnership with <a href="/wiki/NASA" title="NASA">NASA</a> and its Mars entry, descent, and landing technologies research projects.<sup class="reference" id="cite_ref-40"><a href="#cite_note-40">[33]</a></sup> <small>(<a href="#Maiden_flight_of_v1.1">more details below</a>)</small>
</td></tr>
<tr>
<th rowspan="2" scope="row" style="text-align:center;">7
</th>
<td>3 December 2013,<br/>22:41<sup class="reference" id="cite_ref-sfn_wwls20130624_41-0"><a href="#cite_note-sfn_wwls20130624-41">[34]</a></sup>
</td>
<td><a href="/wiki/Falcon_9_v1.1" title="Falcon 9 v1.1">F9 v1.1</a><br/>B1004
</td>
<td><a href="/wiki/Cape_Canaveral_Space_Force_Station" title="Cape Canaveral Space Force Station">CCAFS</a>,<br/><a href="/wiki/Cape_Canaveral_Space_Launch_Complex_40" title="Cape Canaveral Space Launch Complex 40">SLC-40</a>
</td>
<td><a href="/wiki/SES-8" title="SES-8">SES-8</a><sup class="reference" id="cite_ref-sxManifest20120925_28-3"><a href="#cite_note-sxManifest20120925-28">[22]</a></sup><sup class="reference" id="cite_ref-spx-pr_42-0"><a href="#cite_note-spx-pr-42">[35]</a></sup><sup class="reference" id="cite_ref-aw20110323_43-0"><a href="#cite_note-aw20110323-43">[36]</a></sup>
</td>
<td>3,170 kg (6,990 lb)
</td>
<td><a href="/wiki/Geostationary_transfer_orbit" title="Geostationary transfer orbit">GTO</a>
</td>
<td><a href="/wiki/SES_S.A." title="SES S.A.">SES</a>
</td>
<td class="table-success" style="background: LightGreen; color: black; vertical-align: middle; text-align: center;">Success<sup class="reference" id="cite_ref-SNMissionStatus7_44-0"><a href="#cite_note-SNMissionStatus7-44">[37]</a></sup>
</td>
<td class="table-noAttempt" style="background: #ececec; color: black; vertical-align: middle; white-space: nowrap; text-align: center;">No attempt<br/><sup class="reference" id="cite_ref-sf10120131203_45-0"><a href="#cite_note-sf10120131203-45">[38]</a></sup>
</td></tr>
<tr>
<td colspan="9">First <a href="/wiki/Geostationary_transfer_orbit" title="Geostationary transfer orbit">Geostationary transfer orbit</a> (GTO) launch for Falcon 9,<sup class="reference" id="cite_ref-spx-pr_42-1"><a href="#cite_note-spx-pr-42">[35]</a></sup> and first successful reignition of the second stage.<sup class="reference" id="cite_ref-46"><a href="#cite_note-46">[39]</a></sup> SES-8 was inserted into a <a href="/wiki/Geostationary_transfer_orbit" title="Geostationary transfer orbit">Super-Synchronous Transfer Orbit</a> of 79,341 km (49,300 mi) in apogee with an <a href="/wiki/Orbital_inclination" title="Orbital inclination">inclination</a> of 20.55° to the <a href="/wiki/Equator" title="Equator">equator</a>.
</td></tr></tbody></table>
###Markdown
Next, iterate through the heading elements and apply the provided extract_column_from_header() to extract column name one by one
###Code
column_names = []
rows = first_launch_table.find_all('th')
for r in range(len(rows)):
name = extract_column_from_header(rows[r])
if name is not None and len(name)>0:
column_names.append(name)
print(column_names)
###Output
['Flight No.', 'Date and time ( )', 'Launch site', 'Payload', 'Payload mass', 'Orbit', 'Customer', 'Launch outcome']
###Markdown
Create a data frame by parsing the launch HTML tables
###Code
launch_dict = dict.fromkeys(column_names)
# Remove an irrelevent column
del launch_dict['Date and time ( )']
# Initializing dict with each empty value
launch_dict['Flight No.'] = []
launch_dict['Launch site'] = []
launch_dict['Payload'] = []
launch_dict['Payload mass'] = []
launch_dict['Orbit'] = []
launch_dict['Customer'] = []
launch_dict['Launch outcome'] = []
launch_dict['Version Booster'] = []
launch_dict['Booster Landing'] = []
launch_dict['Date'] = []
launch_dict['Time'] = []
###Output
_____no_output_____
###Markdown
Usually, HTML tables in Wiki pages are likely to contain unexpected annotations and other types of noises, such as reference links B0004.1[8], missing values N/A [e], inconsistent formatting, etc.
###Code
extracted_row = 0
#Extract each table
for table_number,table in enumerate(soup.find_all('table',"wikitable plainrowheaders collapsible")):
# get table row
for rows in table.find_all("tr"):
#check to see if first table heading is as number corresponding to launch a number
if rows.th:
if rows.th.string:
flight_number=rows.th.string.strip()
flag=flight_number.isdigit()
else:
flag=False
#get table element
row=rows.find_all('td')
#if it is number save cells in a dictonary
if flag:
extracted_row += 1
# Flight Number value
launch_dict['Flight No.'].append(flight_number)
datatimelist=date_time(row[0])
# Date value
date = datatimelist[0].strip(',')
launch_dict['Date'].append(date)
# Time value
time = datatimelist[1]
launch_dict['Time'].append(time)
# Booster version
bv = booster_version(row[1])
launch_dict['Version Booster'].append(bv)
if not(bv):
bv = row[1].a.string
# Launch Site
launch_site = row[2].a.string
launch_dict['Launch site'].append(launch_site)
# Payload
payload = row[3].a.string
launch_dict['Payload'].append(payload)
# Payload Mass
payload_mass = get_mass(row[4])
launch_dict['Payload mass'].append(payload_mass)
# Orbit
orbit = row[5].a.string
launch_dict['Orbit'].append(orbit)
# Customer
# An unfortunate error format for row 106 requires me to brute force this a bit, no big issue
if extracted_row == 106:
customer = 'Various'
else:
customer = row[6].a.string
launch_dict['Customer'].append(customer)
# Launch outcome
launch_outcome = list(row[7].strings)[0]
launch_dict['Launch outcome'].append(launch_outcome)
# Booster landing
booster_landing = landing_status(row[8])
launch_dict['Booster Landing'].append(booster_landing)
###Output
_____no_output_____
###Markdown
Creating a dataframe from dic.
###Code
df = pd.DataFrame.from_dict(launch_dict, orient='index')
df=df.transpose()
df.head()
###Output
_____no_output_____
###Markdown
Exporting the Data
###Code
df.to_csv('Data/dataset_webscraped.csv', index=False)
###Output
_____no_output_____ |
2. TL Baseline - EfficientNet.ipynb | ###Markdown
Augmentation
###Code
import imgaug.augmenters as iaa
seq = iaa.Sequential([
iaa.SomeOf((0,2),[
iaa.Identity(),
iaa.AverageBlur(k=((3, 5), (5, 7))),
iaa.Rotate((-90,90)),
iaa.Affine(scale=(0.5, 0.95)),
iaa.Multiply((0.50, 1.1))
#,iaa.BlendAlphaRegularGrid(nb_rows=(4, 6), nb_cols=(1, 4),
# foreground=iaa.Multiply(0.0))
#,iaa.Cartoon()
,iaa.Cutout(nb_iterations=(1, 3), size=0.2, squared=False, cval=0)
,iaa.Affine(shear=(-48, 48))
,iaa.Affine(translate_px={"x": (-42, 42), "y": (-36, 36)})
,iaa.KeepSizeByResize(iaa.Resize({"height": (0.70, 0.90), "width": (0.70, 0.90)}))
,iaa.CropAndPad(percent=(-0.2, 0.2))
,iaa.PiecewiseAffine(scale=(0.01, 0.05))
,iaa.PerspectiveTransform(scale=(0.01, 0.1))
,iaa.WithPolarWarping(iaa.CropAndPad(percent=(-0.1, 0.1)))
,iaa.ElasticTransformation(alpha=(0, 3.0), sigma=0.5)
])
#,iaa.SaveDebugImageEveryNBatches(folder_path, 100)
], random_order=True)
def generator(features, labels, batch_size):
while True:
# Fill arrays of batch size with augmented data taken randomly from full passed arrays
indexes = random.sample(range(len(features)), batch_size)
# Transform X and y
x_aug = seq(images =features[indexes])
yield np.array(x_aug), np.array(labels[indexes])
images = generator(data_x,data_y, 9)
image = next(images)
plt.figure(figsize=(10, 10))
for i in range(9):
ax = plt.subplot(3, 3, i + 1)
#augmented_image = seq(images = x_train[:1])
plt.imshow(image[0][i])
plt.title(str(image[1][i]))
plt.axis("off")
###Output
Clipping input data to the valid range for imshow with RGB data ([0..1] for floats or [0..255] for integers).
Clipping input data to the valid range for imshow with RGB data ([0..1] for floats or [0..255] for integers).
###Markdown
Transfer Learning
###Code
x_train, x_val, y_train, y_val = train_test_split(data_x, data_y, test_size=0.30, random_state=42)
y_train = np.argmax(y_train,axis=1)
y_val = np.argmax(y_val,axis=1)
test_x, test_id = load_data("Test")
base_model = applications.EfficientNetB0(
weights='imagenet',
input_shape=(image_size, image_size, 3),
include_top=False)
# Create new model on top.
inputs = keras.Input(shape=(image_size, image_size, 3))
x = base_model(inputs, training=False)
x = keras.layers.GlobalAveragePooling2D()(x)
outputs = keras.layers.Dense(4)(x)
model = keras.Model(inputs, outputs)
model.summary()
# Warm up head
adam = optimizers.Adam(learning_rate=0.0005)
#lr_scheduler = tf.keras.callbacks.LearningRateScheduler(scheduler)
reduce_lr = ReduceLROnPlateau(monitor='val_loss',
factor=0.2,
patience=3,
min_lr=0.00005)
early_stop = EarlyStopping(
monitor='val_loss',
min_delta=0,
patience=6,
verbose=0,
mode='auto',
baseline=None,
restore_best_weights=True
)
batch_size = 64
#num_warmup_steps = 5
# freeze pretrained weights
model.layers[1].trainable = False
model.compile(optimizer=adam,
loss=tf.keras.losses.SparseCategoricalCrossentropy(),
metrics=[tf.keras.metrics.SparseCategoricalCrossentropy()])
history = model.fit(generator(x_train, y_train, batch_size),
shuffle=True,
validation_data = (x_val, y_val),
callbacks = [reduce_lr,early_stop],
epochs=100,
steps_per_epoch=len(x_train)/batch_size ,
verbose=True
)
print(model.optimizer.lr)
# Train entire network
adam = optimizers.Adam(learning_rate=0.0001)
#lr_scheduler = tf.keras.callbacks.LearningRateScheduler(scheduler)
reduce_lr = ReduceLROnPlateau(monitor='val_accuracy',
factor=0.2,
patience=5 ,
min_lr=0.00001)
early_stop = EarlyStopping(
monitor='val_accuracy',
min_delta=0,
patience=10,
verbose=0,
mode='auto',
baseline=None,
restore_best_weights=True
)
# freeze pretrained weights
model.layers[1].trainable = True
model.compile(optimizer=adam,
loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True),
metrics=['accuracy'])
history2 = model.fit(generator(x_train, y_train, batch_size),
shuffle=True,
validation_data = (x_val, y_val),
callbacks = [reduce_lr,early_stop],
epochs=200,
steps_per_epoch=len(x_train)/batch_size ,
verbose=True
)
model = keras.models.load_model('models/efficientNet_9368')
maxIter = 3
iter = 0
# Train entire network
adam = optimizers.Adam(learning_rate=0.0001)
#lr_scheduler = tf.keras.callbacks.LearningRateScheduler(scheduler)
reduce_lr = ReduceLROnPlateau(monitor='val_accuracy',
factor=0.2,
patience=5 ,
min_lr=0.00001)
early_stop = EarlyStopping(
monitor='val_accuracy',
min_delta=0,
patience=10,
verbose=0,
mode='auto',
baseline=None,
restore_best_weights=True
)
model.compile(optimizer=adam,
loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True),
metrics=['accuracy'])
while iter < maxIter:
test_y = model.predict(test_x)
test_pred = tf.nn.softmax(test_y).numpy()
pred_label = np.argmax(test_pred, axis=1)
merged_data_x = np.vstack((x_train, test_x))
merged_data_y = np.vstack((y_train.reshape(-1,1), pred_label.reshape(-1,1)))
history2 = model.fit(generator(merged_data_x, merged_data_y, batch_size),
shuffle=True,
validation_data = (x_val, y_val),
callbacks = [reduce_lr,early_stop],
epochs=200,
steps_per_epoch=len(merged_data_x)/batch_size ,
verbose=True
)
#try kaggle
test_pred = tf.nn.softmax(test_y).numpy()
test_set = np.hstack((test_id, test_pred))
test_set = test_set[test_set[:,1].astype('uint16').argsort()]
test_DF = pd.DataFrame(test_set, index=test_set[:,1], columns=["image_id","id","healthy","multiple_diseases","rust","scab"])
csv_file = f'submissions/efficientNet{iter}.csv'
test_DF[["image_id","healthy","multiple_diseases","rust","scab"]].to_csv(csv_file, index=False)
comments = f'"efficientNet iter {iter} 20210206"'
!kaggle competitions submit -f $csv_file -m$comments plant-pathology-2020-fgvc7
!kaggle competitions submissions plant-pathology-2020-fgvc7
model.save(f'models/efficientNet_{iter}')
iter += 1
model.save('models/efficientNet_9368')
from sklearn.metrics import confusion_matrix
import seaborn as sn
import pandas as pd
import matplotlib.pyplot as plt
labels = ['healthy', 'multiple_diseases', 'rust', 'scab']
y_val_pred = np.argmax(model.predict(x_val), axis=1)
mat = confusion_matrix(y_val, y_val_pred)
df_cm = pd.DataFrame(mat, index = [i for i in labels],
columns = [i for i in labels])
plt.figure(figsize = (10,7))
sn.heatmap(df_cm, annot=True)
# Train on all data before inference
model.fit(generator(x_val, y_val, batch_size),
epochs=2,
steps_per_epoch=len(x_train)/batch_size ,
verbose=True
)
from sklearn.metrics import confusion_matrix
import seaborn as sn
import pandas as pd
import matplotlib.pyplot as plt
labels = ['healthy', 'multiple_diseases', 'rust', 'scab']
y_val_pred = np.argmax(model.predict(x_val), axis=1)
mat = confusion_matrix(y_val, y_val_pred)
df_cm = pd.DataFrame(mat, index = [i for i in labels],
columns = [i for i in labels])
plt.figure(figsize = (10,7))
sn.heatmap(df_cm, annot=True)
# Load test data and their filename for submission file
#test_x, test_id = load_data("Test")
test_y = model.predict(test_x)
test_pred = tf.nn.softmax(test_y).numpy()
test_set = np.hstack((test_id, test_pred))
test_set = test_set[test_set[:,1].astype('uint16').argsort()]
test_DF = pd.DataFrame(test_set, index=test_set[:,1], columns=["image_id","id","healthy","multiple_diseases","rust","scab"])
test_DF[["image_id","healthy","multiple_diseases","rust","scab"]].to_csv('submissions/efficientNet.csv', index=False)
!kaggle competitions submit -f 'submissions/efficientNet.csv' -m"efficientNet baseline 20210130" plant-pathology-2020-fgvc7
!kaggle competitions submissions plant-pathology-2020-fgvc7
###Output
fileName date description status publicScore privateScore
--------------------- ------------------- ---------------------------------- -------- ----------- ------------
efficientNet.csv 2021-02-07 05:12:58 efficientNet baseline 20210130 complete 0.93478 0.93531
efficientNet.csv 2021-02-07 03:07:58 efficientNet baseline 20210130 complete 0.94182 0.93685
EfficientNetB6.csv 2021-02-06 20:03:18 EfficientNetB6 baseline 2021-02-06 complete 0.94181 0.92909
EfficientNetB5.csv 2021-02-06 15:51:55 EfficientNetB5 baseline 2021-02-06 complete 0.94710 0.93022
EfficientNetB4.csv 2021-02-06 13:32:05 EfficientNetB4 baseline 2021-02-06 complete 0.93628 0.93089
EfficientNetB3.csv 2021-02-06 11:32:30 EfficientNetB3 baseline 2021-02-06 complete 0.93135 0.91675
EfficientNetB2.csv 2021-02-06 10:28:00 EfficientNetB2 baseline 2021-02-06 complete 0.92274 0.92275
EfficientNetB1.csv 2021-02-06 09:28:56 EfficientNetB1 baseline 2021-02-06 complete 0.94027 0.93291
EfficientNetB0.csv 2021-02-06 08:36:55 EfficientNetB0 baseline 2021-02-06 complete 0.93026 0.92324
xception.csv 2021-02-06 07:19:59 xception baseline 20210130 complete 0.94455 0.91202
xception.csv 2021-02-06 04:18:08 xception baseline 2021-02-06 complete 0.88840 0.87141
NASNet.csv 2021-01-31 19:49:33 NAXNet baseline 20210131 complete 0.91386 0.90567
xception.csv 2021-01-31 16:12:55 xception baseline 20210130 complete 0.88840 0.87141
inceptionResNetv2.csv 2021-01-31 02:36:15 inception baseline 20210130 complete 0.92493 0.93380
###Markdown
0.9338 is 801 on leaderboard Convert to TFLite
###Code
# Convert the model
converter = tf.lite.TFLiteConverter.from_saved_model("models/xception") # path to the SavedModel directory
tflite_model = converter.convert()
# Save the model.
with open('models/xception.tflite', 'wb') as f:
f.write(tflite_model)
import cv2
for i in range(5):
index = np.random.randint(len(x_train))
print(f"====TF Model result{index}====")
print(model.predict(np.expand_dims(x_train[index],axis=0)))
print(y_train[index])
interpreter = tf.lite.Interpreter(model_path="models/xception.tflite")
interpreter.allocate_tensors()
# Get input and output tensors.
input_details = interpreter.get_input_details()
output_details = interpreter.get_output_details()
# Test the model on random input data.
input_shape = input_details[0]['shape']
img = cv2.resize(x_train[index], (image_size,image_size)).astype('float32')
input_data = np.expand_dims(img, axis = 0)
interpreter.set_tensor(input_details[0]['index'], input_data)
interpreter.invoke()
# The function `get_tensor()` returns a copy of the tensor data.
# Use `tensor()` in order to get a pointer to the tensor.
output_data = interpreter.get_tensor(output_details[0]['index'])
print("====TFLite result====")
print(output_data)
print(np.argmax(output_data))
###Output
====TF Model result118====
[[-5.5913553 0.10580774 -4.789981 10.428146 ]]
3
====TFLite result====
[[-6.151669 0.3389328 -5.203408 11.086818 ]]
3
====TF Model result529====
[[-1.854001 -1.206874 5.5994477 -3.6847982]]
2
====TFLite result====
[[-4.0978193 -1.6762551 9.454673 -6.213326 ]]
2
====TF Model result860====
[[-2.7240992 -1.0839779 5.8567367 -2.9417467]]
2
====TFLite result====
[[-5.7429442 -2.8852344 11.931892 -5.623218 ]]
2
====TF Model result691====
[[-2.3256586 0.12409084 3.7397826 -1.7892648 ]]
2
====TFLite result====
[[-5.9245753 -1.6625859 10.673811 -4.3376884]]
2
====TF Model result1246====
[[ 5.682509 -2.1599436 -2.9038138 -3.121694 ]]
0
====TFLite result====
[[ 5.380403 -2.773593 -2.660941 -4.1204834]]
0
|
3.clustering-pca/1.L1000_pca_clustering_analysis.ipynb | ###Markdown
- PCA and Clustering for L1000 Level-4 profiles (per dose treament) - Use Silhouette and Davies Bouldin scores to assess the number of clusters from K-Means - Use BIC scores to assess the number of clusters from Gaussian Mixture Models (GMM)[reference](https://sites.northwestern.edu/msia/2016/12/08/k-means-shouldnt-be-our-only-choice/)[refeerences](https://gdcoder.com/silhouette-analysis-vs-elbow-method-vs-davies-bouldin-index-selecting-the-optimal-number-of-clusters-for-kmeans-clustering/)
###Code
from sklearn.decomposition import PCA
from sklearn.preprocessing import StandardScaler
import scipy.cluster.hierarchy as shc
from sklearn.metrics import pairwise_distances
from sklearn.cluster import KMeans, AgglomerativeClustering
from scipy.cluster.hierarchy import linkage, dendrogram, fcluster
from sklearn.metrics import silhouette_score
from sklearn.metrics import davies_bouldin_score
from sklearn.mixture import GaussianMixture as GMM
import os
import pathlib
import pandas as pd
import numpy as np
import re
from os import walk
from collections import Counter
import random
import matplotlib.pyplot as plt
%matplotlib inline
import seaborn as sns
sns.set_style("darkgrid")
##sns.set_palette(["red", "green", "orange","blue","gray","purple"])
sns.set_context("talk")
import warnings
warnings.simplefilter(action='ignore', category=FutureWarning)
np.warnings.filterwarnings('ignore', category=np.VisibleDeprecationWarning)
number_of_pcs = 300
L1000_level4_path = "../1.Data-exploration/Profiles_level4/L1000/L1000_lvl4_cpd_replicate_datasets"
output_path = "results/L1000/"
# Load common compounds
common_file = pathlib.Path(
"..", "6.paper_figures", "data", "significant_compounds_by_threshold_both_assays.tsv.gz"
)
common_df = pd.read_csv(common_file, sep="\t")
common_compounds = common_df.compound.unique()
print(len(common_compounds))
print(common_df.shape)
common_df.head()
# Load level 4 compounds
df_level4 = pd.read_csv(os.path.join(L1000_level4_path, 'L1000_level4_cpd_replicates.csv.gz'),
compression='gzip',low_memory = False)
df_level4.pert_iname = df_level4.pert_iname.str.lower()
df_level4 = df_level4.query("pert_iname in @common_compounds").reset_index(drop=True)
print(df_level4.pert_iname.nunique())
print(df_level4.shape)
df_level4.head(2)
def save_to_csv(df, path, file_name, compress=None):
"""saves dataframes to csv"""
if not os.path.exists(path):
os.mkdir(path)
df.to_csv(os.path.join(path, file_name), index=False, compression=compress)
def extract_dose_df(df, dose_num):
"""Extract data for each treatment dose"""
df_dose = df[df['dose'] == dose_num].reset_index(drop=True)
metadata_cols = ['replicate_id', 'sig_id', 'pert_id', 'pert_idose', 'det_plate',
'det_well', 'dose', 'Metadata_broad_sample', 'moa', 'pert_iname']
df_dose.drop(metadata_cols, axis = 1, inplace = True)
return df_dose
def transform_pca(df, dose_num, no_of_pcs=350):
"""Perform PCA Analysis"""
scaler = StandardScaler()
scaled_agg = scaler.fit_transform(df)
df_scaled = pd.DataFrame(data = scaled_agg, columns = ['feat_' + str(x) for x in range(1,df.shape[1]+1)])
#lets extract features with the most variance in our dataset
pca = PCA(n_components=no_of_pcs)
pc = pca.fit_transform(scaled_agg)
df_pc = pd.DataFrame(data = pc, columns = ['PC' + str(x) for x in range(1,no_of_pcs+1)])
df_pc['dose'] = dose_num
#Plotting the Cumulative Summation of the Explained Variance
plt.figure(figsize=(16, 8))
fig = plt.plot(np.cumsum(pca.explained_variance_ratio_))
plt.xlabel('Number of Components')
plt.ylabel('Cumulative Explained Variance')
plt.title('Explained Variance by Principal Components')
plt.xticks(np.arange(0, no_of_pcs+1, step=20))
plt.show()
return pca, df_pc, df_scaled
def SelBest(arr:list, X:int)->list:
'''
returns the set of X configurations with shorter distance
'''
dx=np.argsort(arr)[:X]
return arr[dx]
def calc_bic(pc_data, no_of_clusters=40):
"""
Computes Bayesian Information Criteria scores (BIC) when Gaussian Mixture Models (GMM) is fitted on a data
to assess the clustering on the data
"""
n_clusters=np.arange(2, no_of_clusters+1)
bics=[]
bics_err=[]
iterations=1
for n in n_clusters:
#print(n)
tmp_bic=[]
for _ in range(iterations):
gmm=GMM(n, n_init=2, max_iter=1000,
tol=1e-4,init_params='kmeans').fit(pc_data)
tmp_bic.append(gmm.bic(pc_data))
val=np.mean(SelBest(np.array(tmp_bic), int(iterations/1)))
err=np.std(tmp_bic)
bics.append(val)
bics_err.append(err)
return bics, bics_err
def plot_bics(bics):
plt.figure(figsize=(14,6))
plt.plot(list(bics.keys()), list(bics.values()), label='BIC')
plt.title("BIC Scores", fontsize=20)
plt.xlabel("N. of clusters")
plt.ylabel("Score")
plt.legend()
def calculate_score(df, no_of_clusters=40):
"""
Assess K-means clustering using Silhoutte and Davies bouldin scores
"""
silh_score = {}
davie_score = {}
for k in range(2, no_of_clusters+1):
kmeans = KMeans(n_clusters=k,
max_iter=1000,
tol=1e-4)
label = kmeans.fit_predict(df)
silhouette_avg = silhouette_score(df, label)
davie_avg = davies_bouldin_score(df,label)
silh_score[k] = silhouette_avg
davie_score[k] = davie_avg
#print("For n_clusters={}, The average silhouette_score is: {}".format(k, silhouette_avg))
#print("For n_clusters={}, The davies_bouldin_score is: {}".format(k, davie_avg))
return silh_score, davie_score
def plot_score(score, score_name):
plt.rcParams.update({'font.size': 12})
plt.figure(figsize=(12, 6))
plt.plot(list(score.keys()), list(score.values()),
linestyle='--', marker='o', color='orange')
plt.title(f"{score_name} across clusters", fontsize=20)
plt.xlabel("Number of clusters")
plt.ylabel(score_name)
plt.xticks(np.arange(0, max(list(score.keys()))+1, step=2))
plt.show()
def create_df(data_dict, col_name, dose_num):
df = pd.DataFrame(data_dict.items(), columns = ['cluster', col_name])
df['dose'] = dose_num
return df
###Output
_____no_output_____
###Markdown
Calculate Silhouette scores in only the common compounds
###Code
metadata_cols = [
'replicate_id', 'sig_id', 'pert_id', 'pert_idose', 'det_plate',
'det_well', 'Metadata_broad_sample', 'moa', 'pert_iname'
]
# Extract only the features (drop metadata)
df_common_alldose = df_level4.drop(metadata_cols, axis = 1)
df_common_alldose = df_common_alldose.loc[:, df_common_alldose.columns.str.endswith("_at")]
print(df_common_alldose.shape)
pca_all_thresh, df_pc_all_thresh, df_scaled_all_thresh = transform_pca(
df_common_alldose, dose_num=None, no_of_pcs=number_of_pcs
)
np.sum(pca_all_thresh.explained_variance_ratio_)
def plot_pca_var(pca, pc_num):
#plt.rcParams.update({'font.size': 12})
plt.figure(figsize = (14, 8))
df_var = pd.DataFrame({'var':pca.explained_variance_ratio_, 'PC':['PC' + str(x) for x in range(1,pc_num+1)]})
df_var['var'] = df_var['var'] * 100
#sns.barplot(x='PC',y="var", data=df_var, color="c")
plt.bar(df_var['PC'], df_var['var'], color ='c')
plt.xlim(0, pc_num+1)
plt.ylabel('Explained Variance %')
plt.xlabel('Principal Components')
plt.xticks(np.arange(0, pc_num, step=20))
plt.title("Amount of variance explained by each Principal component for Cell painting level-4 profiles")
##plt.savefig('cluster_images/var_exp_PCA.png')
plt.show()
return df_var
df_var_full = plot_pca_var(pca_all_thresh, number_of_pcs)
save_to_csv(df_var_full, output_path, 'L1000_pca_explained_variance.csv')
doseall_thresh_silh_score, doseall_thresh_davie_score = calculate_score(df_pc_all_thresh.drop(['dose'], axis = 1))
df_silhall_thresh = create_df(doseall_thresh_silh_score, 'Average_silhouette_score', "common_compounds")
df_dball_thresh = create_df(doseall_thresh_davie_score, 'davies_bouldin_score', "common_compounds")
# bics_dose_all, _ = calc_bic(df_pc_all_thresh.drop(['dose'], axis = 1))
# dose_bic_score_all = {idx+2:score for idx, score in enumerate(bics_dose_all)}
# df_bics_dose_all = create_df(dose_bic_score_all, 'BIC_score', "common_compounds")
plot_score(doseall_thresh_silh_score, 'Average Silhouette')
plot_score(doseall_thresh_davie_score, 'Davies Bouldin score')
# Output to file
output_file = pathlib.Path("results/L1000/L1000_silhouette_scores_compounds_common_compounds.csv")
df_silhall_thresh.to_csv(output_file, index=False)
output_file = pathlib.Path("results/L1000/L1000_davies_compounds_common_compounds.csv")
df_dball_thresh.to_csv(output_file, index=False)
# output_file = pathlib.Path("results/L1000/L1000_bic_compounds_common_compounds.csv")
# df_bics_dose_all.to_csv(output_file, index=False)
###Output
_____no_output_____
###Markdown
Perform the same analysis within each dose separately
###Code
silh_list = []
db_list = []
bic_list = []
for dose in [1, 2, 3, 4, 5, 6]:
print(f"Now analyzing dose {dose}")
df_dose = extract_dose_df(df_level4, dose_num=dose)
pca_dose, df_pc_dose, df_scaled_dose = transform_pca(df_dose, dose_num=dose)
print(np.sum(pca_dose.explained_variance_ratio_))
# Calculate BIC
bics_dose, _ = calc_bic(df_pc_dose.drop(['dose'], axis = 1))
dose_bic_score = {idx+2:score for idx, score in enumerate(bics_dose)}
# Calculate Silhouette and Davies Boulding index
dose_silh_score, dose_davie_score = calculate_score(df_pc_dose.drop(['dose'], axis = 1))
# Save output
df_silh = create_df(dose_silh_score, 'Average_silhouette_score', dose)
silh_list.append(df_silh)
df_db = create_df(dose_davie_score, 'davies_bouldin_score', dose)
db_list.append(df_db)
df_bic = create_df(dose_bic_score, 'BIC_score', dose)
bic_list.append(df_bic)
df_silh = pd.concat(silh_list, ignore_index=True)
df_db = pd.concat(db_list, ignore_index=True)
df_bic = pd.concat(bic_list, ignore_index=True)
save_to_csv(df_silh, output_path, 'L1000_silhouette_scores.csv')
save_to_csv(df_db, output_path, 'L1000_db_scores.csv')
save_to_csv(df_bic, output_path, 'L1000_bic_scores.csv')
###Output
_____no_output_____ |
sentiment-analysis-network.ipynb | ###Markdown
Sentiment-Analysis-Networkby Qiren Sun 1.Get reviews and labels
###Code
def pretty_print_review_and_label(i):
print(labels[i]+'\t:\t' + reviews[i])
#Get reiews & labels
g=open('reviews.txt','r')
reviews=list(map(lambda x: x[:-1],g.readlines()))
g.close()
g=open('labels.txt','r')
labels=list(map(lambda x: x[:-1].upper(),g.readlines()))
g.close()
pretty_print_review_and_label(1)
###Output
NEGATIVE : story of a man who has unnatural feelings for a pig . starts out with a opening scene that is a terrific example of absurd comedy . a formal orchestra audience is turned into an insane violent mob by the crazy chantings of it s singers . unfortunately it stays absurd the whole time with no general narrative eventually making it just too off putting . even those from the era should be turned off . the cryptic dialogue would make shakespeare seem easy to a third grader . on a technical level it s better than you might think with some good cinematography by future great vilmos zsigmond . future stars sally kirkland and frederic forrest can be seen briefly .
###Markdown
2.Building a Neural Network Note: This part includes training and testing data, reducing neural noise, getting better weight initialization and improving the training speed.
###Code
import time
import sys
import numpy as np
from collections import Counter
class SentimentNetwork:
def __init__(self,reviews,labels,min_count=10,polarity_cutoff=0.1,hidden_nodes=10,learning_rate=0.1):
np.random.seed(1)
self.pre_process_data(reviews,labels,polarity_cutoff,min_count)
self.init_network(len(self.review_vocab),hidden_nodes,1,learning_rate)
def pre_process_data(self,reviews,labels,polarity_cutoff,min_count):
##---------------------------------------
#Calculate positive-to-negative ratios for words before building vocabulary
#
positive_count=Counter()
negative_count=Counter()
total_count=Counter()
for i in range(len(reviews)):
if(labels[i]=='POSITIVE'):
for word in reviews[i].split(' '):
positive_count[word]+=1
total_count[word]+=1
else:
for word in reviews[i].split(' '):
negative_count[word]+=1
total_count[word]+=1
pos_neg_radio=Counter()
for term,cnt in list(total_count.most_common()):
if (cnt>=100):
pos_neg_radios=positive_count[term]/float(negative_count[term]+1)
pos_neg_radio[term]=pos_neg_radios
for word,radio in pos_neg_radio.most_common():
if(radio>1):
pos_neg_radio[word]=np.log(radio)
else:
pos_neg_radio[word]=-np.log(1/(radio+0.01))
#
##------------------------------------------
# populate review_vocab with all of the words in the given reviews
review_vocab=set()
for review in reviews:
for word in review.split(' '):
if(total_count[word]>min_count):
if(word in pos_neg_radio.keys()):
if(pos_neg_radio[word]>=polarity_cutoff) or (pos_neg_radio[word]<=-polarity_cutoff):
review_vocab.add(word)
else:
review_vocab.add(word)
# Convert the vocabulary set to a list so we can access words via indices
self.review_vocab=list(review_vocab)
label_vocab=set()
for label in labels:
label_vocab.add(label)
self.label_vocab=list(label_vocab)
self.review_vocab_size = len(self.review_vocab)
self.label_vocab_size = len(self.label_vocab)
# Create a dictionary of words in the vocabulary mapped to index positions
self.word2index=dict()
for i,word in enumerate(self.review_vocab):
self.word2index[word]=i
# Create a dictionary of labels mapped to index positions
self.label2index=dict()
for i,label in enumerate(self.label_vocab):
self.label2index[label]=i
def init_network(self,input_nodes,hidden_nodes,output_nodes,learning_rate):
# Set number of nodes in input, hidden and output layers.
self.input_nodes=input_nodes
self.hidden_nodes=hidden_nodes
self.output_nodes=output_nodes
self.learning_rate=learning_rate
# Initialize weights
self.weights_0_1=np.zeros((self.input_nodes,self.hidden_nodes))
self.weights_1_2=np.random.normal(0.0, self.hidden_nodes**-.5, (self.hidden_nodes,self.output_nodes))
# The input layer, a two-dimensional matrix with shape 1 x hidden_nodes
self.layer_1=np.zeros((1,hidden_nodes))
def get_target_for_label(self,label):
if(label == 'POSITIVE'):
return 1
else:
return 0
def sigmoid(self,x):
return 1 / (1 + np.exp(-x))
def sigmoid_output_2_derivative(self,output):
return output * (1 - output)
def train(self, training_reviews_raw, training_labels):
#pre-process training reviews so we can deal directly with the indices of non-zero inputs
training_reviews=list()
for review in training_reviews_raw:
indices=set()
for word in review.split(' '):
if (word in self.word2index.keys()):
indices.add(self.word2index[word])
training_reviews.append(list(indices))
# make sure out we have a matching number of reviews and labels
#assert(len(training_reviews) == len(training_labels))
# Keep track of correct predictions to display accuracy during training
correct_so_far = 0
# Remember when we started for printing time statistics
start = time.time()
# loop through all the given reviews and run a forward and backward pass,
# updating weights for every item
for i in range(len(training_reviews)):
# Get the next review and its correct label
review = training_reviews[i]
label = training_labels[i]
#hidden layer
#Add in only the weights for non-zero items
self.layer_1 *= 0
for index in review:
self.layer_1 += self.weights_0_1[index]
#output layer
layer_2 = self.sigmoid(self.layer_1.dot(self.weights_1_2))
# Output error
# Output layer error is the difference between desired target and actual output.
layer_2_error = layer_2 - self.get_target_for_label(label)
layer_2_error_term = layer_2_error * self.sigmoid_output_2_derivative(layer_2)
# Backpropagated error
layer_1_error = layer_2_error_term.dot(self.weights_1_2.T) # errors propagated to the hidden layer
layer_1_error_term = layer_1_error # hidden layer gradients -linear
# Update the weights
self.weights_1_2 -= self.layer_1.T.dot(layer_2_error_term) * self.learning_rate
for ind in review:
self.weights_0_1[ind]-= layer_1_error_term[0]*self.learning_rate
# Keep track of correct predictions.
if(layer_2 >= 0.5 and label == 'POSITIVE'):
correct_so_far += 1
elif(layer_2 < 0.5 and label == 'NEGATIVE'):
correct_so_far += 1
# For debug purposes, print out our prediction accuracy and speed
# throughout the training process.
elapsed_time = float(time.time() - start)
reviews_per_second = i / elapsed_time if elapsed_time > 0 else 0
sys.stdout.write("\rProgress:" + str(100 * i/float(len(training_reviews)))[:4] \
+ "% Speed(reviews/sec):" + str(reviews_per_second)[0:5] \
+ " #Correct:" + str(correct_so_far) + " #Trained:" + str(i+1) \
+ " Training Accuracy:" + str(correct_so_far * 100 / float(i+1))[:4] + "%")
if(i % 2500 == 0):
print('')
def test(self,testing_reviews,testing_labels):
# keep track of how many correct predictions we make
correct = 0
# we'll time how many predictions per second we make
start = time.time()
# Loop through each of the given reviews and call run to predict
# its label.
for i in range(len(testing_reviews)):
pred = self.run(testing_reviews[i])
if(pred == testing_labels[i]):
correct += 1
# For debug purposes, print out our prediction accuracy and speed
# throughout the prediction process.
elapsed_time = float(time.time() - start)
reviews_per_second = i / elapsed_time if elapsed_time > 0 else 0
sys.stdout.write("\rProgress:" + str(100 * i/float(len(testing_reviews)))[:4] \
+ "% Speed(reviews/sec):" + str(reviews_per_second)[0:5] \
+ " #Correct:" + str(correct) + " #Tested:" + str(i+1) \
+ " Testing Accuracy:" + str(correct * 100 / float(i+1))[:4] + "%")
def run(self,review):
#hidden layer
self.layer_1 *= 0
unique_indices = set()
for word in review.lower().split(" "):
if word in self.word2index.keys():
unique_indices.add(self.word2index[word])
for index in unique_indices:
self.layer_1 += self.weights_0_1[index]
#output layer
layer_2=self.sigmoid(self.layer_1.dot(self.weights_1_2))
if(layer_2[0] >= 0.5):
return "POSITIVE"
else:
return "NEGATIVE"
###Output
_____no_output_____
###Markdown
3.Training
###Code
mlp = SentimentNetwork(reviews[:-1000],labels[:-1000],min_count=20,polarity_cutoff=0.2,learning_rate=0.01)
mlp.train(reviews[:-1000],labels[:-1000])
###Output
Progress:0.0% Speed(reviews/sec):0.0 #Correct:1 #Trained:1 Training Accuracy:100.%
Progress:10.4% Speed(reviews/sec):1387. #Correct:2027 #Trained:2501 Training Accuracy:81.0%
Progress:20.8% Speed(reviews/sec):1295. #Correct:4090 #Trained:5001 Training Accuracy:81.7%
Progress:31.2% Speed(reviews/sec):1282. #Correct:6235 #Trained:7501 Training Accuracy:83.1%
Progress:41.6% Speed(reviews/sec):1276. #Correct:8381 #Trained:10001 Training Accuracy:83.8%
Progress:52.0% Speed(reviews/sec):1284. #Correct:10537 #Trained:12501 Training Accuracy:84.2%
Progress:62.5% Speed(reviews/sec):1282. #Correct:12683 #Trained:15001 Training Accuracy:84.5%
Progress:72.9% Speed(reviews/sec):1289. #Correct:14839 #Trained:17501 Training Accuracy:84.7%
Progress:83.3% Speed(reviews/sec):1279. #Correct:17030 #Trained:20001 Training Accuracy:85.1%
Progress:93.7% Speed(reviews/sec):1275. #Correct:19230 #Trained:22501 Training Accuracy:85.4%
Progress:99.9% Speed(reviews/sec):1271. #Correct:20554 #Trained:24000 Training Accuracy:85.6%
###Markdown
4.Testing
###Code
mlp.test(reviews[-1000:],labels[-1000:])
###Output
Progress:0.0% Speed(reviews/sec):0 #Correct:1 #Tested:1 Testing Accuracy:100.%
Progress:0.1% Speed(reviews/sec):997.6 #Correct:2 #Tested:2 Testing Accuracy:100.%
Progress:0.2% Speed(reviews/sec):1995. #Correct:2 #Tested:3 Testing Accuracy:66.6%
Progress:0.3% Speed(reviews/sec):1496. #Correct:3 #Tested:4 Testing Accuracy:75.0%
Progress:0.4% Speed(reviews/sec):1994. #Correct:4 #Tested:5 Testing Accuracy:80.0%
Progress:0.5% Speed(reviews/sec):2493. #Correct:5 #Tested:6 Testing Accuracy:83.3%
Progress:0.6% Speed(reviews/sec):1994. #Correct:6 #Tested:7 Testing Accuracy:85.7%
Progress:0.7% Speed(reviews/sec):2326. #Correct:7 #Tested:8 Testing Accuracy:87.5%
Progress:0.8% Speed(reviews/sec):1994. #Correct:8 #Tested:9 Testing Accuracy:88.8%
Progress:0.9% Speed(reviews/sec):1795. #Correct:9 #Tested:10 Testing Accuracy:90.0%
Progress:1.0% Speed(reviews/sec):1994. #Correct:10 #Tested:11 Testing Accuracy:90.9%
Progress:1.1% Speed(reviews/sec):1828. #Correct:11 #Tested:12 Testing Accuracy:91.6%
Progress:1.2% Speed(reviews/sec):1994. #Correct:12 #Tested:13 Testing Accuracy:92.3%
Progress:1.3% Speed(reviews/sec):2160. #Correct:13 #Tested:14 Testing Accuracy:92.8%
Progress:1.4% Speed(reviews/sec):1994. #Correct:14 #Tested:15 Testing Accuracy:93.3%
Progress:1.5% Speed(reviews/sec):2137. #Correct:15 #Tested:16 Testing Accuracy:93.7%
Progress:1.6% Speed(reviews/sec):1994. #Correct:16 #Tested:17 Testing Accuracy:94.1%
Progress:1.7% Speed(reviews/sec):1883. #Correct:17 #Tested:18 Testing Accuracy:94.4%
Progress:1.8% Speed(reviews/sec):1994. #Correct:18 #Tested:19 Testing Accuracy:94.7%
Progress:1.9% Speed(reviews/sec):2105. #Correct:18 #Tested:20 Testing Accuracy:90.0%
Progress:2.0% Speed(reviews/sec):1994. #Correct:19 #Tested:21 Testing Accuracy:90.4%
Progress:2.1% Speed(reviews/sec):2094. #Correct:20 #Tested:22 Testing Accuracy:90.9%
Progress:2.2% Speed(reviews/sec):1994. #Correct:21 #Tested:23 Testing Accuracy:91.3%
Progress:2.3% Speed(reviews/sec):2085. #Correct:22 #Tested:24 Testing Accuracy:91.6%
Progress:2.4% Speed(reviews/sec):1994. #Correct:23 #Tested:25 Testing Accuracy:92.0%
Progress:2.5% Speed(reviews/sec):1917. #Correct:24 #Tested:26 Testing Accuracy:92.3%
Progress:2.6% Speed(reviews/sec):1994. #Correct:25 #Tested:27 Testing Accuracy:92.5%
Progress:2.7% Speed(reviews/sec):1795. #Correct:26 #Tested:28 Testing Accuracy:92.8%
Progress:2.8% Speed(reviews/sec):1861. #Correct:27 #Tested:29 Testing Accuracy:93.1%
Progress:2.9% Speed(reviews/sec):1928. #Correct:28 #Tested:30 Testing Accuracy:93.3%
Progress:3.0% Speed(reviews/sec):1870. #Correct:29 #Tested:31 Testing Accuracy:93.5%
Progress:3.1% Speed(reviews/sec):1932. #Correct:30 #Tested:32 Testing Accuracy:93.7%
Progress:3.2% Speed(reviews/sec):1877. #Correct:31 #Tested:33 Testing Accuracy:93.9%
Progress:3.3% Speed(reviews/sec):1935. #Correct:32 #Tested:34 Testing Accuracy:94.1%
Progress:3.4% Speed(reviews/sec):1883. #Correct:33 #Tested:35 Testing Accuracy:94.2%
Progress:3.5% Speed(reviews/sec):1939. #Correct:34 #Tested:36 Testing Accuracy:94.4%
Progress:3.6% Speed(reviews/sec):1889. #Correct:35 #Tested:37 Testing Accuracy:94.5%
Progress:3.7% Speed(reviews/sec):1942. #Correct:36 #Tested:38 Testing Accuracy:94.7%
Progress:3.8% Speed(reviews/sec):1894. #Correct:37 #Tested:39 Testing Accuracy:94.8%
Progress:3.9% Speed(reviews/sec):1852. #Correct:38 #Tested:40 Testing Accuracy:95.0%
Progress:4.0% Speed(reviews/sec):1899. #Correct:39 #Tested:41 Testing Accuracy:95.1%
Progress:4.1% Speed(reviews/sec):1858. #Correct:40 #Tested:42 Testing Accuracy:95.2%
Progress:4.2% Speed(reviews/sec):1821. #Correct:41 #Tested:43 Testing Accuracy:95.3%
Progress:4.3% Speed(reviews/sec):1786. #Correct:42 #Tested:44 Testing Accuracy:95.4%
Progress:4.4% Speed(reviews/sec):1755. #Correct:43 #Tested:45 Testing Accuracy:95.5%
Progress:4.5% Speed(reviews/sec):1726. #Correct:44 #Tested:46 Testing Accuracy:95.6%
Progress:4.6% Speed(reviews/sec):1699. #Correct:45 #Tested:47 Testing Accuracy:95.7%
Progress:4.7% Speed(reviews/sec):1736. #Correct:46 #Tested:48 Testing Accuracy:95.8%
Progress:4.8% Speed(reviews/sec):1709. #Correct:47 #Tested:49 Testing Accuracy:95.9%
Progress:4.9% Speed(reviews/sec):1745. #Correct:48 #Tested:50 Testing Accuracy:96.0%
Progress:5.0% Speed(reviews/sec):1719. #Correct:49 #Tested:51 Testing Accuracy:96.0%
Progress:5.1% Speed(reviews/sec):1753. #Correct:50 #Tested:52 Testing Accuracy:96.1%
Progress:5.2% Speed(reviews/sec):1728. #Correct:51 #Tested:53 Testing Accuracy:96.2%
Progress:5.3% Speed(reviews/sec):1705. #Correct:52 #Tested:54 Testing Accuracy:96.2%
Progress:5.4% Speed(reviews/sec):1737. #Correct:53 #Tested:55 Testing Accuracy:96.3%
Progress:5.5% Speed(reviews/sec):1769. #Correct:54 #Tested:56 Testing Accuracy:96.4%
Progress:5.6% Speed(reviews/sec):1692. #Correct:55 #Tested:57 Testing Accuracy:96.4%
Progress:5.7% Speed(reviews/sec):1722. #Correct:56 #Tested:58 Testing Accuracy:96.5%
Progress:5.8% Speed(reviews/sec):1752. #Correct:57 #Tested:59 Testing Accuracy:96.6%
Progress:5.9% Speed(reviews/sec):1730. #Correct:58 #Tested:60 Testing Accuracy:96.6%
Progress:6.0% Speed(reviews/sec):1760. #Correct:58 #Tested:61 Testing Accuracy:95.0%
Progress:6.1% Speed(reviews/sec):1738. #Correct:59 #Tested:62 Testing Accuracy:95.1%
Progress:6.2% Speed(reviews/sec):1766. #Correct:60 #Tested:63 Testing Accuracy:95.2%
Progress:6.3% Speed(reviews/sec):1745. #Correct:61 #Tested:64 Testing Accuracy:95.3%
Progress:6.4% Speed(reviews/sec):1772. #Correct:61 #Tested:65 Testing Accuracy:93.8%
Progress:6.5% Speed(reviews/sec):1752. #Correct:61 #Tested:66 Testing Accuracy:92.4%
Progress:6.6% Speed(reviews/sec):1779. #Correct:62 #Tested:67 Testing Accuracy:92.5%
Progress:6.7% Speed(reviews/sec):1758. #Correct:63 #Tested:68 Testing Accuracy:92.6%
Progress:6.8% Speed(reviews/sec):1784. #Correct:64 #Tested:69 Testing Accuracy:92.7%
Progress:6.9% Speed(reviews/sec):1764. #Correct:65 #Tested:70 Testing Accuracy:92.8%
Progress:7.0% Speed(reviews/sec):1790. #Correct:65 #Tested:71 Testing Accuracy:91.5%
Progress:7.1% Speed(reviews/sec):1770. #Correct:66 #Tested:72 Testing Accuracy:91.6%
Progress:7.2% Speed(reviews/sec):1795. #Correct:67 #Tested:73 Testing Accuracy:91.7%
Progress:7.3% Speed(reviews/sec):1820. #Correct:68 #Tested:74 Testing Accuracy:91.8%
Progress:7.4% Speed(reviews/sec):1844. #Correct:68 #Tested:75 Testing Accuracy:90.6%
Progress:7.5% Speed(reviews/sec):1824. #Correct:69 #Tested:76 Testing Accuracy:90.7%
Progress:7.6% Speed(reviews/sec):1848. #Correct:70 #Tested:77 Testing Accuracy:90.9%
Progress:7.7% Speed(reviews/sec):1828. #Correct:71 #Tested:78 Testing Accuracy:91.0%
Progress:7.8% Speed(reviews/sec):1852. #Correct:72 #Tested:79 Testing Accuracy:91.1%
Progress:7.9% Speed(reviews/sec):1832. #Correct:73 #Tested:80 Testing Accuracy:91.2%
Progress:8.0% Speed(reviews/sec):1855. #Correct:74 #Tested:81 Testing Accuracy:91.3%
Progress:8.1% Speed(reviews/sec):1795. #Correct:74 #Tested:82 Testing Accuracy:90.2%
Progress:8.2% Speed(reviews/sec):1817. #Correct:75 #Tested:83 Testing Accuracy:90.3%
Progress:8.3% Speed(reviews/sec):1799. #Correct:76 #Tested:84 Testing Accuracy:90.4%
Progress:8.4% Speed(reviews/sec):1821. #Correct:77 #Tested:85 Testing Accuracy:90.5%
Progress:8.5% Speed(reviews/sec):1803. #Correct:78 #Tested:86 Testing Accuracy:90.6%
Progress:8.6% Speed(reviews/sec):1824. #Correct:79 #Tested:87 Testing Accuracy:90.8%
Progress:8.7% Speed(reviews/sec):1770. #Correct:79 #Tested:88 Testing Accuracy:89.7%
Progress:8.8% Speed(reviews/sec):1791. #Correct:80 #Tested:89 Testing Accuracy:89.8%
Progress:8.9% Speed(reviews/sec):1775. #Correct:81 #Tested:90 Testing Accuracy:90.0%
Progress:9.0% Speed(reviews/sec):1760. #Correct:82 #Tested:91 Testing Accuracy:90.1%
Progress:9.1% Speed(reviews/sec):1779. #Correct:83 #Tested:92 Testing Accuracy:90.2%
Progress:9.2% Speed(reviews/sec):1764. #Correct:84 #Tested:93 Testing Accuracy:90.3%
Progress:9.3% Speed(reviews/sec):1783. #Correct:85 #Tested:94 Testing Accuracy:90.4%
Progress:9.4% Speed(reviews/sec):1802. #Correct:86 #Tested:95 Testing Accuracy:90.5%
Progress:9.5% Speed(reviews/sec):1787. #Correct:87 #Tested:96 Testing Accuracy:90.6%
Progress:9.6% Speed(reviews/sec):1773. #Correct:88 #Tested:97 Testing Accuracy:90.7%
Progress:9.7% Speed(reviews/sec):1791. #Correct:89 #Tested:98 Testing Accuracy:90.8%
Progress:9.8% Speed(reviews/sec):1809. #Correct:90 #Tested:99 Testing Accuracy:90.9%
Progress:9.9% Speed(reviews/sec):1795. #Correct:91 #Tested:100 Testing Accuracy:91.0%
Progress:10.0% Speed(reviews/sec):1813. #Correct:92 #Tested:101 Testing Accuracy:91.0%
Progress:10.1% Speed(reviews/sec):1798. #Correct:93 #Tested:102 Testing Accuracy:91.1%
Progress:10.2% Speed(reviews/sec):1816. #Correct:94 #Tested:103 Testing Accuracy:91.2%
Progress:10.3% Speed(reviews/sec):1802. #Correct:94 #Tested:104 Testing Accuracy:90.3%
Progress:10.4% Speed(reviews/sec):1788. #Correct:95 #Tested:105 Testing Accuracy:90.4%
Progress:10.5% Speed(reviews/sec):1805. #Correct:96 #Tested:106 Testing Accuracy:90.5%
Progress:10.6% Speed(reviews/sec):1791. #Correct:97 #Tested:107 Testing Accuracy:90.6%
Progress:10.7% Speed(reviews/sec):1808. #Correct:98 #Tested:108 Testing Accuracy:90.7%
Progress:10.8% Speed(reviews/sec):1825. #Correct:99 #Tested:109 Testing Accuracy:90.8%
Progress:10.9% Speed(reviews/sec):1811. #Correct:99 #Tested:110 Testing Accuracy:90.0%
Progress:11.0% Speed(reviews/sec):1828. #Correct:100 #Tested:111 Testing Accuracy:90.0%
Progress:11.1% Speed(reviews/sec):1814. #Correct:101 #Tested:112 Testing Accuracy:90.1%
Progress:11.2% Speed(reviews/sec):1831. #Correct:102 #Tested:113 Testing Accuracy:90.2%
Progress:11.3% Speed(reviews/sec):1817. #Correct:103 #Tested:114 Testing Accuracy:90.3%
Progress:11.4% Speed(reviews/sec):1804. #Correct:104 #Tested:115 Testing Accuracy:90.4%
Progress:11.5% Speed(reviews/sec):1792. #Correct:104 #Tested:116 Testing Accuracy:89.6%
Progress:11.6% Speed(reviews/sec):1807. #Correct:105 #Tested:117 Testing Accuracy:89.7%
Progress:11.7% Speed(reviews/sec):1794. #Correct:105 #Tested:118 Testing Accuracy:88.9%
Progress:11.8% Speed(reviews/sec):1810. #Correct:106 #Tested:119 Testing Accuracy:89.0%
Progress:11.9% Speed(reviews/sec):1798. #Correct:107 #Tested:120 Testing Accuracy:89.1%
Progress:12.0% Speed(reviews/sec):1813. #Correct:108 #Tested:121 Testing Accuracy:89.2%
Progress:12.1% Speed(reviews/sec):1828. #Correct:109 #Tested:122 Testing Accuracy:89.3%
Progress:12.2% Speed(reviews/sec):1816. #Correct:110 #Tested:123 Testing Accuracy:89.4%
Progress:12.3% Speed(reviews/sec):1803. #Correct:111 #Tested:124 Testing Accuracy:89.5%
Progress:12.4% Speed(reviews/sec):1791. #Correct:112 #Tested:125 Testing Accuracy:89.6%
Progress:12.5% Speed(reviews/sec):1779. #Correct:113 #Tested:126 Testing Accuracy:89.6%
Progress:12.6% Speed(reviews/sec):1794. #Correct:114 #Tested:127 Testing Accuracy:89.7%
Progress:12.7% Speed(reviews/sec):1783. #Correct:115 #Tested:128 Testing Accuracy:89.8%
Progress:12.8% Speed(reviews/sec):1798. #Correct:116 #Tested:129 Testing Accuracy:89.9%
Progress:12.9% Speed(reviews/sec):1786. #Correct:117 #Tested:130 Testing Accuracy:90.0%
Progress:13.0% Speed(reviews/sec):1800. #Correct:117 #Tested:131 Testing Accuracy:89.3%
Progress:13.1% Speed(reviews/sec):1814. #Correct:118 #Tested:132 Testing Accuracy:89.3%
Progress:13.2% Speed(reviews/sec):1803. #Correct:119 #Tested:133 Testing Accuracy:89.4%
Progress:13.3% Speed(reviews/sec):1817. #Correct:120 #Tested:134 Testing Accuracy:89.5%
Progress:13.4% Speed(reviews/sec):1806. #Correct:121 #Tested:135 Testing Accuracy:89.6%
Progress:13.5% Speed(reviews/sec):1819. #Correct:122 #Tested:136 Testing Accuracy:89.7%
Progress:13.6% Speed(reviews/sec):1807. #Correct:123 #Tested:137 Testing Accuracy:89.7%
Progress:13.7% Speed(reviews/sec):1820. #Correct:124 #Tested:138 Testing Accuracy:89.8%
Progress:13.8% Speed(reviews/sec):1834. #Correct:125 #Tested:139 Testing Accuracy:89.9%
Progress:13.9% Speed(reviews/sec):1800. #Correct:126 #Tested:140 Testing Accuracy:90.0%
Progress:14.0% Speed(reviews/sec):1813. #Correct:127 #Tested:141 Testing Accuracy:90.0%
Progress:14.1% Speed(reviews/sec):1826. #Correct:128 #Tested:142 Testing Accuracy:90.1%
Progress:14.2% Speed(reviews/sec):1815. #Correct:129 #Tested:143 Testing Accuracy:90.2%
Progress:14.3% Speed(reviews/sec):1828. #Correct:130 #Tested:144 Testing Accuracy:90.2%
Progress:14.4% Speed(reviews/sec):1817. #Correct:131 #Tested:145 Testing Accuracy:90.3%
Progress:14.5% Speed(reviews/sec):1830. #Correct:132 #Tested:146 Testing Accuracy:90.4%
Progress:14.6% Speed(reviews/sec):1819. #Correct:133 #Tested:147 Testing Accuracy:90.4%
Progress:14.7% Speed(reviews/sec):1809. #Correct:134 #Tested:148 Testing Accuracy:90.5%
Progress:14.8% Speed(reviews/sec):1822. #Correct:135 #Tested:149 Testing Accuracy:90.6%
Progress:14.9% Speed(reviews/sec):1811. #Correct:136 #Tested:150 Testing Accuracy:90.6%
Progress:15.0% Speed(reviews/sec):1823. #Correct:137 #Tested:151 Testing Accuracy:90.7%
Progress:15.1% Speed(reviews/sec):1813. #Correct:138 #Tested:152 Testing Accuracy:90.7%
Progress:15.2% Speed(reviews/sec):1803. #Correct:139 #Tested:153 Testing Accuracy:90.8%
Progress:15.3% Speed(reviews/sec):1795. #Correct:140 #Tested:154 Testing Accuracy:90.9%
Progress:15.4% Speed(reviews/sec):1806. #Correct:141 #Tested:155 Testing Accuracy:90.9%
Progress:15.5% Speed(reviews/sec):1797. #Correct:142 #Tested:156 Testing Accuracy:91.0%
Progress:15.6% Speed(reviews/sec):1788. #Correct:143 #Tested:157 Testing Accuracy:91.0%
Progress:15.7% Speed(reviews/sec):1779. #Correct:144 #Tested:158 Testing Accuracy:91.1%
Progress:15.8% Speed(reviews/sec):1790. #Correct:144 #Tested:159 Testing Accuracy:90.5%
Progress:15.9% Speed(reviews/sec):1781. #Correct:145 #Tested:160 Testing Accuracy:90.6%
Progress:16.0% Speed(reviews/sec):1792. #Correct:145 #Tested:161 Testing Accuracy:90.0%
Progress:16.1% Speed(reviews/sec):1784. #Correct:146 #Tested:162 Testing Accuracy:90.1%
Progress:16.2% Speed(reviews/sec):1795. #Correct:147 #Tested:163 Testing Accuracy:90.1%
Progress:16.3% Speed(reviews/sec):1786. #Correct:148 #Tested:164 Testing Accuracy:90.2%
Progress:16.4% Speed(reviews/sec):1797. #Correct:149 #Tested:165 Testing Accuracy:90.3%
Progress:16.5% Speed(reviews/sec):1788. #Correct:150 #Tested:166 Testing Accuracy:90.3%
Progress:16.6% Speed(reviews/sec):1780. #Correct:151 #Tested:167 Testing Accuracy:90.4%
Progress:16.7% Speed(reviews/sec):1771. #Correct:152 #Tested:168 Testing Accuracy:90.4%
Progress:16.8% Speed(reviews/sec):1782. #Correct:153 #Tested:169 Testing Accuracy:90.5%
Progress:16.9% Speed(reviews/sec):1793. #Correct:154 #Tested:170 Testing Accuracy:90.5%
Progress:17.0% Speed(reviews/sec):1784. #Correct:154 #Tested:171 Testing Accuracy:90.0%
Progress:17.1% Speed(reviews/sec):1795. #Correct:155 #Tested:172 Testing Accuracy:90.1%
Progress:17.2% Speed(reviews/sec):1786. #Correct:156 #Tested:173 Testing Accuracy:90.1%
Progress:17.3% Speed(reviews/sec):1778. #Correct:157 #Tested:174 Testing Accuracy:90.2%
Progress:17.4% Speed(reviews/sec):1770. #Correct:158 #Tested:175 Testing Accuracy:90.2%
Progress:17.5% Speed(reviews/sec):1780. #Correct:159 #Tested:176 Testing Accuracy:90.3%
Progress:17.6% Speed(reviews/sec):1773. #Correct:160 #Tested:177 Testing Accuracy:90.3%
Progress:17.7% Speed(reviews/sec):1783. #Correct:161 #Tested:178 Testing Accuracy:90.4%
Progress:17.8% Speed(reviews/sec):1775. #Correct:162 #Tested:179 Testing Accuracy:90.5%
Progress:17.9% Speed(reviews/sec):1785. #Correct:163 #Tested:180 Testing Accuracy:90.5%
Progress:18.0% Speed(reviews/sec):1777. #Correct:164 #Tested:181 Testing Accuracy:90.6%
Progress:18.1% Speed(reviews/sec):1769. #Correct:165 #Tested:182 Testing Accuracy:90.6%
Progress:18.2% Speed(reviews/sec):1779. #Correct:166 #Tested:183 Testing Accuracy:90.7%
Progress:18.3% Speed(reviews/sec):1789. #Correct:167 #Tested:184 Testing Accuracy:90.7%
Progress:18.4% Speed(reviews/sec):1764. #Correct:168 #Tested:185 Testing Accuracy:90.8%
Progress:18.5% Speed(reviews/sec):1774. #Correct:169 #Tested:186 Testing Accuracy:90.8%
Progress:18.6% Speed(reviews/sec):1766. #Correct:169 #Tested:187 Testing Accuracy:90.3%
Progress:18.7% Speed(reviews/sec):1776. #Correct:170 #Tested:188 Testing Accuracy:90.4%
Progress:18.8% Speed(reviews/sec):1768. #Correct:170 #Tested:189 Testing Accuracy:89.9%
Progress:18.9% Speed(reviews/sec):1778. #Correct:171 #Tested:190 Testing Accuracy:90.0%
Progress:19.0% Speed(reviews/sec):1770. #Correct:172 #Tested:191 Testing Accuracy:90.0%
Progress:19.1% Speed(reviews/sec):1780. #Correct:173 #Tested:192 Testing Accuracy:90.1%
Progress:19.2% Speed(reviews/sec):1789. #Correct:173 #Tested:193 Testing Accuracy:89.6%
Progress:19.3% Speed(reviews/sec):1782. #Correct:173 #Tested:194 Testing Accuracy:89.1%
Progress:19.4% Speed(reviews/sec):1775. #Correct:174 #Tested:195 Testing Accuracy:89.2%
Progress:19.5% Speed(reviews/sec):1784. #Correct:174 #Tested:196 Testing Accuracy:88.7%
Progress:19.6% Speed(reviews/sec):1777. #Correct:175 #Tested:197 Testing Accuracy:88.8%
Progress:19.7% Speed(reviews/sec):1786. #Correct:176 #Tested:198 Testing Accuracy:88.8%
Progress:19.8% Speed(reviews/sec):1779. #Correct:177 #Tested:199 Testing Accuracy:88.9%
Progress:19.9% Speed(reviews/sec):1788. #Correct:178 #Tested:200 Testing Accuracy:89.0%
Progress:20.0% Speed(reviews/sec):1796. #Correct:179 #Tested:201 Testing Accuracy:89.0%
Progress:20.1% Speed(reviews/sec):1789. #Correct:180 #Tested:202 Testing Accuracy:89.1%
Progress:20.2% Speed(reviews/sec):1782. #Correct:181 #Tested:203 Testing Accuracy:89.1%
Progress:20.3% Speed(reviews/sec):1791. #Correct:182 #Tested:204 Testing Accuracy:89.2%
Progress:20.4% Speed(reviews/sec):1784. #Correct:183 #Tested:205 Testing Accuracy:89.2%
Progress:20.5% Speed(reviews/sec):1793. #Correct:184 #Tested:206 Testing Accuracy:89.3%
Progress:20.6% Speed(reviews/sec):1802. #Correct:185 #Tested:207 Testing Accuracy:89.3%
Progress:20.7% Speed(reviews/sec):1795. #Correct:186 #Tested:208 Testing Accuracy:89.4%
Progress:20.8% Speed(reviews/sec):1803. #Correct:187 #Tested:209 Testing Accuracy:89.4%
Progress:20.9% Speed(reviews/sec):1796. #Correct:187 #Tested:210 Testing Accuracy:89.0%
Progress:21.0% Speed(reviews/sec):1790. #Correct:187 #Tested:211 Testing Accuracy:88.6%
Progress:21.1% Speed(reviews/sec):1798. #Correct:188 #Tested:212 Testing Accuracy:88.6%
Progress:21.2% Speed(reviews/sec):1807. #Correct:189 #Tested:213 Testing Accuracy:88.7%
Progress:21.3% Speed(reviews/sec):1800. #Correct:190 #Tested:214 Testing Accuracy:88.7%
Progress:21.4% Speed(reviews/sec):1793. #Correct:191 #Tested:215 Testing Accuracy:88.8%
Progress:21.5% Speed(reviews/sec):1786. #Correct:192 #Tested:216 Testing Accuracy:88.8%
Progress:21.6% Speed(reviews/sec):1795. #Correct:193 #Tested:217 Testing Accuracy:88.9%
Progress:21.7% Speed(reviews/sec):1803. #Correct:194 #Tested:218 Testing Accuracy:88.9%
Progress:21.8% Speed(reviews/sec):1796. #Correct:195 #Tested:219 Testing Accuracy:89.0%
Progress:21.9% Speed(reviews/sec):1805. #Correct:196 #Tested:220 Testing Accuracy:89.0%
Progress:22.0% Speed(reviews/sec):1798. #Correct:197 #Tested:221 Testing Accuracy:89.1%
Progress:22.1% Speed(reviews/sec):1791. #Correct:198 #Tested:222 Testing Accuracy:89.1%
Progress:22.2% Speed(reviews/sec):1800. #Correct:199 #Tested:223 Testing Accuracy:89.2%
Progress:22.3% Speed(reviews/sec):1793. #Correct:200 #Tested:224 Testing Accuracy:89.2%
Progress:22.4% Speed(reviews/sec):1787. #Correct:200 #Tested:225 Testing Accuracy:88.8%
Progress:22.5% Speed(reviews/sec):1780. #Correct:201 #Tested:226 Testing Accuracy:88.9%
Progress:22.6% Speed(reviews/sec):1774. #Correct:202 #Tested:227 Testing Accuracy:88.9%
Progress:22.7% Speed(reviews/sec):1782. #Correct:203 #Tested:228 Testing Accuracy:89.0%
Progress:22.8% Speed(reviews/sec):1776. #Correct:204 #Tested:229 Testing Accuracy:89.0%
Progress:22.9% Speed(reviews/sec):1784. #Correct:205 #Tested:230 Testing Accuracy:89.1%
Progress:23.0% Speed(reviews/sec):1778. #Correct:206 #Tested:231 Testing Accuracy:89.1%
Progress:23.1% Speed(reviews/sec):1785. #Correct:207 #Tested:232 Testing Accuracy:89.2%
Progress:23.2% Speed(reviews/sec):1779. #Correct:208 #Tested:233 Testing Accuracy:89.2%
Progress:23.3% Speed(reviews/sec):1787. #Correct:209 #Tested:234 Testing Accuracy:89.3%
Progress:23.4% Speed(reviews/sec):1781. #Correct:210 #Tested:235 Testing Accuracy:89.3%
Progress:23.5% Speed(reviews/sec):1789. #Correct:210 #Tested:236 Testing Accuracy:88.9%
Progress:23.6% Speed(reviews/sec):1783. #Correct:211 #Tested:237 Testing Accuracy:89.0%
Progress:23.7% Speed(reviews/sec):1777. #Correct:212 #Tested:238 Testing Accuracy:89.0%
Progress:23.8% Speed(reviews/sec):1784. #Correct:213 #Tested:239 Testing Accuracy:89.1%
Progress:23.9% Speed(reviews/sec):1778. #Correct:214 #Tested:240 Testing Accuracy:89.1%
Progress:24.0% Speed(reviews/sec):1773. #Correct:215 #Tested:241 Testing Accuracy:89.2%
Progress:24.1% Speed(reviews/sec):1780. #Correct:215 #Tested:242 Testing Accuracy:88.8%
Progress:24.2% Speed(reviews/sec):1774. #Correct:216 #Tested:243 Testing Accuracy:88.8%
Progress:24.3% Speed(reviews/sec):1782. #Correct:217 #Tested:244 Testing Accuracy:88.9%
Progress:24.4% Speed(reviews/sec):1776. #Correct:218 #Tested:245 Testing Accuracy:88.9%
Progress:24.5% Speed(reviews/sec):1783. #Correct:219 #Tested:246 Testing Accuracy:89.0%
Progress:24.6% Speed(reviews/sec):1777. #Correct:220 #Tested:247 Testing Accuracy:89.0%
Progress:24.7% Speed(reviews/sec):1785. #Correct:221 #Tested:248 Testing Accuracy:89.1%
Progress:24.8% Speed(reviews/sec):1792. #Correct:222 #Tested:249 Testing Accuracy:89.1%
Progress:24.9% Speed(reviews/sec):1799. #Correct:222 #Tested:250 Testing Accuracy:88.8%
Progress:25.0% Speed(reviews/sec):1793. #Correct:223 #Tested:251 Testing Accuracy:88.8%
Progress:25.1% Speed(reviews/sec):1800. #Correct:224 #Tested:252 Testing Accuracy:88.8%
Progress:25.2% Speed(reviews/sec):1808. #Correct:225 #Tested:253 Testing Accuracy:88.9%
Progress:25.3% Speed(reviews/sec):1802. #Correct:226 #Tested:254 Testing Accuracy:88.9%
Progress:25.4% Speed(reviews/sec):1809. #Correct:227 #Tested:255 Testing Accuracy:89.0%
Progress:25.5% Speed(reviews/sec):1816. #Correct:228 #Tested:256 Testing Accuracy:89.0%
Progress:25.6% Speed(reviews/sec):1823. #Correct:229 #Tested:257 Testing Accuracy:89.1%
Progress:25.7% Speed(reviews/sec):1817. #Correct:230 #Tested:258 Testing Accuracy:89.1%
Progress:25.8% Speed(reviews/sec):1824. #Correct:230 #Tested:259 Testing Accuracy:88.8%
Progress:25.9% Speed(reviews/sec):1831. #Correct:230 #Tested:260 Testing Accuracy:88.4%
Progress:26.0% Speed(reviews/sec):1839. #Correct:231 #Tested:261 Testing Accuracy:88.5%
Progress:26.1% Speed(reviews/sec):1833. #Correct:232 #Tested:262 Testing Accuracy:88.5%
Progress:26.2% Speed(reviews/sec):1840. #Correct:233 #Tested:263 Testing Accuracy:88.5%
Progress:26.3% Speed(reviews/sec):1834. #Correct:234 #Tested:264 Testing Accuracy:88.6%
Progress:26.4% Speed(reviews/sec):1841. #Correct:235 #Tested:265 Testing Accuracy:88.6%
Progress:26.5% Speed(reviews/sec):1835. #Correct:236 #Tested:266 Testing Accuracy:88.7%
Progress:26.6% Speed(reviews/sec):1829. #Correct:237 #Tested:267 Testing Accuracy:88.7%
Progress:26.7% Speed(reviews/sec):1836. #Correct:237 #Tested:268 Testing Accuracy:88.4%
Progress:26.8% Speed(reviews/sec):1830. #Correct:238 #Tested:269 Testing Accuracy:88.4%
Progress:26.9% Speed(reviews/sec):1837. #Correct:238 #Tested:270 Testing Accuracy:88.1%
Progress:27.0% Speed(reviews/sec):1831. #Correct:238 #Tested:271 Testing Accuracy:87.8%
Progress:27.1% Speed(reviews/sec):1838. #Correct:239 #Tested:272 Testing Accuracy:87.8%
Progress:27.2% Speed(reviews/sec):1832. #Correct:240 #Tested:273 Testing Accuracy:87.9%
Progress:27.3% Speed(reviews/sec):1839. #Correct:241 #Tested:274 Testing Accuracy:87.9%
Progress:27.4% Speed(reviews/sec):1834. #Correct:241 #Tested:275 Testing Accuracy:87.6%
Progress:27.5% Speed(reviews/sec):1828. #Correct:242 #Tested:276 Testing Accuracy:87.6%
Progress:27.6% Speed(reviews/sec):1822. #Correct:243 #Tested:277 Testing Accuracy:87.7%
Progress:27.7% Speed(reviews/sec):1829. #Correct:244 #Tested:278 Testing Accuracy:87.7%
Progress:27.8% Speed(reviews/sec):1836. #Correct:245 #Tested:279 Testing Accuracy:87.8%
Progress:27.9% Speed(reviews/sec):1830. #Correct:246 #Tested:280 Testing Accuracy:87.8%
Progress:28.0% Speed(reviews/sec):1837. #Correct:247 #Tested:281 Testing Accuracy:87.9%
Progress:28.1% Speed(reviews/sec):1831. #Correct:248 #Tested:282 Testing Accuracy:87.9%
Progress:28.2% Speed(reviews/sec):1838. #Correct:249 #Tested:283 Testing Accuracy:87.9%
Progress:28.3% Speed(reviews/sec):1844. #Correct:250 #Tested:284 Testing Accuracy:88.0%
Progress:28.4% Speed(reviews/sec):1839. #Correct:251 #Tested:285 Testing Accuracy:88.0%
Progress:28.5% Speed(reviews/sec):1833. #Correct:252 #Tested:286 Testing Accuracy:88.1%
Progress:28.6% Speed(reviews/sec):1840. #Correct:253 #Tested:287 Testing Accuracy:88.1%
Progress:28.7% Speed(reviews/sec):1846. #Correct:254 #Tested:288 Testing Accuracy:88.1%
Progress:28.8% Speed(reviews/sec):1853. #Correct:255 #Tested:289 Testing Accuracy:88.2%
Progress:28.9% Speed(reviews/sec):1847. #Correct:256 #Tested:290 Testing Accuracy:88.2%
Progress:29.0% Speed(reviews/sec):1854. #Correct:257 #Tested:291 Testing Accuracy:88.3%
Progress:29.1% Speed(reviews/sec):1848. #Correct:258 #Tested:292 Testing Accuracy:88.3%
Progress:29.2% Speed(reviews/sec):1854. #Correct:259 #Tested:293 Testing Accuracy:88.3%
Progress:29.3% Speed(reviews/sec):1861. #Correct:260 #Tested:294 Testing Accuracy:88.4%
Progress:29.4% Speed(reviews/sec):1867. #Correct:261 #Tested:295 Testing Accuracy:88.4%
Progress:29.5% Speed(reviews/sec):1862. #Correct:262 #Tested:296 Testing Accuracy:88.5%
Progress:29.6% Speed(reviews/sec):1868. #Correct:263 #Tested:297 Testing Accuracy:88.5%
Progress:29.7% Speed(reviews/sec):1862. #Correct:263 #Tested:298 Testing Accuracy:88.2%
Progress:29.8% Speed(reviews/sec):1869. #Correct:264 #Tested:299 Testing Accuracy:88.2%
Progress:29.9% Speed(reviews/sec):1863. #Correct:265 #Tested:300 Testing Accuracy:88.3%
Progress:30.0% Speed(reviews/sec):1870. #Correct:266 #Tested:301 Testing Accuracy:88.3%
Progress:30.1% Speed(reviews/sec):1864. #Correct:267 #Tested:302 Testing Accuracy:88.4%
Progress:30.2% Speed(reviews/sec):1847. #Correct:268 #Tested:303 Testing Accuracy:88.4%
Progress:30.3% Speed(reviews/sec):1842. #Correct:269 #Tested:304 Testing Accuracy:88.4%
Progress:30.4% Speed(reviews/sec):1848. #Correct:270 #Tested:305 Testing Accuracy:88.5%
Progress:30.5% Speed(reviews/sec):1854. #Correct:270 #Tested:306 Testing Accuracy:88.2%
Progress:30.6% Speed(reviews/sec):1849. #Correct:271 #Tested:307 Testing Accuracy:88.2%
Progress:30.7% Speed(reviews/sec):1844. #Correct:271 #Tested:308 Testing Accuracy:87.9%
Progress:30.8% Speed(reviews/sec):1850. #Correct:272 #Tested:309 Testing Accuracy:88.0%
Progress:30.9% Speed(reviews/sec):1845. #Correct:273 #Tested:310 Testing Accuracy:88.0%
Progress:31.0% Speed(reviews/sec):1840. #Correct:274 #Tested:311 Testing Accuracy:88.1%
Progress:31.1% Speed(reviews/sec):1846. #Correct:275 #Tested:312 Testing Accuracy:88.1%
Progress:31.2% Speed(reviews/sec):1841. #Correct:276 #Tested:313 Testing Accuracy:88.1%
Progress:31.3% Speed(reviews/sec):1847. #Correct:277 #Tested:314 Testing Accuracy:88.2%
Progress:31.4% Speed(reviews/sec):1842. #Correct:278 #Tested:315 Testing Accuracy:88.2%
Progress:31.5% Speed(reviews/sec):1848. #Correct:279 #Tested:316 Testing Accuracy:88.2%
Progress:31.6% Speed(reviews/sec):1853. #Correct:280 #Tested:317 Testing Accuracy:88.3%
Progress:31.7% Speed(reviews/sec):1848. #Correct:280 #Tested:318 Testing Accuracy:88.0%
Progress:31.8% Speed(reviews/sec):1854. #Correct:281 #Tested:319 Testing Accuracy:88.0%
Progress:31.9% Speed(reviews/sec):1849. #Correct:282 #Tested:320 Testing Accuracy:88.1%
Progress:32.0% Speed(reviews/sec):1855. #Correct:283 #Tested:321 Testing Accuracy:88.1%
Progress:32.1% Speed(reviews/sec):1861. #Correct:283 #Tested:322 Testing Accuracy:87.8%
Progress:32.2% Speed(reviews/sec):1867. #Correct:284 #Tested:323 Testing Accuracy:87.9%
Progress:32.3% Speed(reviews/sec):1862. #Correct:285 #Tested:324 Testing Accuracy:87.9%
Progress:32.4% Speed(reviews/sec):1857. #Correct:286 #Tested:325 Testing Accuracy:88.0%
Progress:32.5% Speed(reviews/sec):1862. #Correct:287 #Tested:326 Testing Accuracy:88.0%
Progress:32.6% Speed(reviews/sec):1868. #Correct:288 #Tested:327 Testing Accuracy:88.0%
Progress:32.7% Speed(reviews/sec):1863. #Correct:289 #Tested:328 Testing Accuracy:88.1%
Progress:32.8% Speed(reviews/sec):1858. #Correct:290 #Tested:329 Testing Accuracy:88.1%
Progress:32.9% Speed(reviews/sec):1864. #Correct:290 #Tested:330 Testing Accuracy:87.8%
Progress:33.0% Speed(reviews/sec):1870. #Correct:291 #Tested:331 Testing Accuracy:87.9%
Progress:33.1% Speed(reviews/sec):1865. #Correct:292 #Tested:332 Testing Accuracy:87.9%
Progress:33.2% Speed(reviews/sec):1870. #Correct:293 #Tested:333 Testing Accuracy:87.9%
Progress:33.3% Speed(reviews/sec):1865. #Correct:294 #Tested:334 Testing Accuracy:88.0%
Progress:33.4% Speed(reviews/sec):1860. #Correct:295 #Tested:335 Testing Accuracy:88.0%
Progress:33.5% Speed(reviews/sec):1866. #Correct:296 #Tested:336 Testing Accuracy:88.0%
Progress:33.6% Speed(reviews/sec):1861. #Correct:297 #Tested:337 Testing Accuracy:88.1%
Progress:33.7% Speed(reviews/sec):1867. #Correct:298 #Tested:338 Testing Accuracy:88.1%
Progress:33.8% Speed(reviews/sec):1872. #Correct:299 #Tested:339 Testing Accuracy:88.2%
Progress:33.9% Speed(reviews/sec):1867. #Correct:300 #Tested:340 Testing Accuracy:88.2%
Progress:34.0% Speed(reviews/sec):1873. #Correct:301 #Tested:341 Testing Accuracy:88.2%
Progress:34.1% Speed(reviews/sec):1868. #Correct:302 #Tested:342 Testing Accuracy:88.3%
Progress:34.2% Speed(reviews/sec):1863. #Correct:303 #Tested:343 Testing Accuracy:88.3%
Progress:34.3% Speed(reviews/sec):1869. #Correct:304 #Tested:344 Testing Accuracy:88.3%
Progress:34.4% Speed(reviews/sec):1864. #Correct:305 #Tested:345 Testing Accuracy:88.4%
Progress:34.5% Speed(reviews/sec):1870. #Correct:306 #Tested:346 Testing Accuracy:88.4%
Progress:34.6% Speed(reviews/sec):1865. #Correct:306 #Tested:347 Testing Accuracy:88.1%
Progress:34.7% Speed(reviews/sec):1870. #Correct:307 #Tested:348 Testing Accuracy:88.2%
Progress:34.8% Speed(reviews/sec):1865. #Correct:308 #Tested:349 Testing Accuracy:88.2%
Progress:34.9% Speed(reviews/sec):1871. #Correct:309 #Tested:350 Testing Accuracy:88.2%
Progress:35.0% Speed(reviews/sec):1876. #Correct:310 #Tested:351 Testing Accuracy:88.3%
Progress:35.1% Speed(reviews/sec):1872. #Correct:311 #Tested:352 Testing Accuracy:88.3%
Progress:35.2% Speed(reviews/sec):1877. #Correct:312 #Tested:353 Testing Accuracy:88.3%
Progress:35.3% Speed(reviews/sec):1882. #Correct:312 #Tested:354 Testing Accuracy:88.1%
Progress:35.4% Speed(reviews/sec):1877. #Correct:313 #Tested:355 Testing Accuracy:88.1%
Progress:35.5% Speed(reviews/sec):1873. #Correct:314 #Tested:356 Testing Accuracy:88.2%
Progress:35.6% Speed(reviews/sec):1878. #Correct:315 #Tested:357 Testing Accuracy:88.2%
Progress:35.7% Speed(reviews/sec):1873. #Correct:315 #Tested:358 Testing Accuracy:87.9%
Progress:35.8% Speed(reviews/sec):1879. #Correct:316 #Tested:359 Testing Accuracy:88.0%
Progress:35.9% Speed(reviews/sec):1874. #Correct:317 #Tested:360 Testing Accuracy:88.0%
Progress:36.0% Speed(reviews/sec):1879. #Correct:318 #Tested:361 Testing Accuracy:88.0%
Progress:36.1% Speed(reviews/sec):1875. #Correct:319 #Tested:362 Testing Accuracy:88.1%
Progress:36.2% Speed(reviews/sec):1870. #Correct:320 #Tested:363 Testing Accuracy:88.1%
Progress:36.3% Speed(reviews/sec):1875. #Correct:321 #Tested:364 Testing Accuracy:88.1%
Progress:36.4% Speed(reviews/sec):1880. #Correct:322 #Tested:365 Testing Accuracy:88.2%
Progress:36.5% Speed(reviews/sec):1876. #Correct:323 #Tested:366 Testing Accuracy:88.2%
Progress:36.6% Speed(reviews/sec):1881. #Correct:324 #Tested:367 Testing Accuracy:88.2%
Progress:36.7% Speed(reviews/sec):1886. #Correct:325 #Tested:368 Testing Accuracy:88.3%
Progress:36.8% Speed(reviews/sec):1882. #Correct:326 #Tested:369 Testing Accuracy:88.3%
Progress:36.9% Speed(reviews/sec):1887. #Correct:327 #Tested:370 Testing Accuracy:88.3%
Progress:37.0% Speed(reviews/sec):1882. #Correct:328 #Tested:371 Testing Accuracy:88.4%
Progress:37.1% Speed(reviews/sec):1878. #Correct:328 #Tested:372 Testing Accuracy:88.1%
Progress:37.2% Speed(reviews/sec):1883. #Correct:328 #Tested:373 Testing Accuracy:87.9%
Progress:37.3% Speed(reviews/sec):1878. #Correct:329 #Tested:374 Testing Accuracy:87.9%
Progress:37.4% Speed(reviews/sec):1883. #Correct:330 #Tested:375 Testing Accuracy:88.0%
Progress:37.5% Speed(reviews/sec):1879. #Correct:331 #Tested:376 Testing Accuracy:88.0%
Progress:37.6% Speed(reviews/sec):1884. #Correct:332 #Tested:377 Testing Accuracy:88.0%
Progress:37.7% Speed(reviews/sec):1889. #Correct:333 #Tested:378 Testing Accuracy:88.0%
Progress:37.8% Speed(reviews/sec):1884. #Correct:333 #Tested:379 Testing Accuracy:87.8%
Progress:37.9% Speed(reviews/sec):1889. #Correct:334 #Tested:380 Testing Accuracy:87.8%
Progress:38.0% Speed(reviews/sec):1885. #Correct:335 #Tested:381 Testing Accuracy:87.9%
Progress:38.1% Speed(reviews/sec):1881. #Correct:336 #Tested:382 Testing Accuracy:87.9%
Progress:38.2% Speed(reviews/sec):1886. #Correct:337 #Tested:383 Testing Accuracy:87.9%
Progress:38.3% Speed(reviews/sec):1881. #Correct:338 #Tested:384 Testing Accuracy:88.0%
Progress:38.4% Speed(reviews/sec):1886. #Correct:338 #Tested:385 Testing Accuracy:87.7%
Progress:38.5% Speed(reviews/sec):1891. #Correct:339 #Tested:386 Testing Accuracy:87.8%
Progress:38.6% Speed(reviews/sec):1887. #Correct:340 #Tested:387 Testing Accuracy:87.8%
Progress:38.7% Speed(reviews/sec):1892. #Correct:340 #Tested:388 Testing Accuracy:87.6%
Progress:38.8% Speed(reviews/sec):1896. #Correct:340 #Tested:389 Testing Accuracy:87.4%
Progress:38.9% Speed(reviews/sec):1892. #Correct:341 #Tested:390 Testing Accuracy:87.4%
Progress:39.0% Speed(reviews/sec):1897. #Correct:342 #Tested:391 Testing Accuracy:87.4%
Progress:39.1% Speed(reviews/sec):1893. #Correct:343 #Tested:392 Testing Accuracy:87.5%
Progress:39.2% Speed(reviews/sec):1897. #Correct:344 #Tested:393 Testing Accuracy:87.5%
Progress:39.3% Speed(reviews/sec):1902. #Correct:345 #Tested:394 Testing Accuracy:87.5%
Progress:39.4% Speed(reviews/sec):1898. #Correct:346 #Tested:395 Testing Accuracy:87.5%
Progress:39.5% Speed(reviews/sec):1893. #Correct:347 #Tested:396 Testing Accuracy:87.6%
Progress:39.6% Speed(reviews/sec):1898. #Correct:348 #Tested:397 Testing Accuracy:87.6%
Progress:39.7% Speed(reviews/sec):1903. #Correct:349 #Tested:398 Testing Accuracy:87.6%
Progress:39.8% Speed(reviews/sec):1899. #Correct:349 #Tested:399 Testing Accuracy:87.4%
Progress:39.9% Speed(reviews/sec):1904. #Correct:350 #Tested:400 Testing Accuracy:87.5%
Progress:40.0% Speed(reviews/sec):1899. #Correct:351 #Tested:401 Testing Accuracy:87.5%
Progress:40.1% Speed(reviews/sec):1895. #Correct:352 #Tested:402 Testing Accuracy:87.5%
Progress:40.2% Speed(reviews/sec):1900. #Correct:353 #Tested:403 Testing Accuracy:87.5%
Progress:40.3% Speed(reviews/sec):1904. #Correct:353 #Tested:404 Testing Accuracy:87.3%
Progress:40.4% Speed(reviews/sec):1900. #Correct:354 #Tested:405 Testing Accuracy:87.4%
Progress:40.5% Speed(reviews/sec):1905. #Correct:355 #Tested:406 Testing Accuracy:87.4%
Progress:40.6% Speed(reviews/sec):1901. #Correct:356 #Tested:407 Testing Accuracy:87.4%
Progress:40.7% Speed(reviews/sec):1905. #Correct:357 #Tested:408 Testing Accuracy:87.5%
Progress:40.8% Speed(reviews/sec):1910. #Correct:358 #Tested:409 Testing Accuracy:87.5%
Progress:40.9% Speed(reviews/sec):1906. #Correct:359 #Tested:410 Testing Accuracy:87.5% |
exercises/2.46.ipynb | ###Markdown
練習問題2.46原点からある⼀点に張られる⼆次元ベクトルは、 x座標とy座標からなるペアとして表現できる。 コンストラクタ make-vectと、それに対応するセレクタxcor-vect, ycor-vectを与え、 ベクトルに対するデータ抽象化を実装せよ。 それらのセレクタとコンストラクタによって、 ベクトルの⾜し算、引き算、スカラによるかけ算という演算を⾏う⼿続き add-vect, sub-vect, scale-vectを実装せよ。 $$(x_1, y_1) + (x_2, y_2) = (x_1 + x_2, y_1 + y_2) \\(x_1, y_1) − (x_2, y_2) = (x_1 − x_2, y_1 − y_2) \\s \cdot (x, y) = (s x, s y) \\$$
###Code
; 回答
; ベクトルコンストラクタ・セレクタ
(define (make-vect x y)(cons x y))
(define (xcor-vect v)(car v))
(define (ycor-vect v)(cdr v))
; ベクトル演算
(define (add-vect v1 v2)
(make-vect (+ (xcor-vect v1) (xcor-vect v2))
(+ (ycor-vect v1) (ycor-vect v2))
)
)
(define (sub-vect v1 v2)
(make-vect (- (xcor-vect v1) (xcor-vect v2))
(- (ycor-vect v1) (ycor-vect v2))
)
)
(define (scale-vect s v)
(make-vect (* s (xcor-vect v)) (* s (ycor-vect v))
)
)
; 動作確認
(define v1 (make-vect 1 -2))
(define v2 (make-vect -3 4))
; 動作確認
(add-vect v1 v2)
; 動作確認
(sub-vect v1 v2)
; 動作確認
(scale-vect 5 v2)
###Output
_____no_output_____ |
Deep_Learning/sentiment_analysis.ipynb | ###Markdown
Sentiment Analysis using a TextBlob
###Code
from textblob import TextBlob
from newspaper import Article
url ='https://www.theatlantic.com/science/archive/2021/08/robert-malone-vaccine-inventor-vaccine-skeptic/619734/?utm_source=pocket-newtab-intl-en'
article=Article(url)
article.download()
article.parse()
article.nlp()
text = article.summary
print(text)
blob = TextBlob(text)
sentiment = blob.sentiment.polarity
""" -1 To 1 (Bad --> Good) """
print(sentiment)
if sentiment == 0:
print("The content of the article looks neutral")
elif (0<sentiment < 0.5 ):
print("The Article is some-what positive")
elif sentiment >= 0.5:
print("The Article is Postive")
elif sentiment <= -0.5:
print("The Article is Negative")
elif (-0.5 < sentiment < 0):
print("The Article is some-what negative ")
###Output
“You’re hearing it from an individual who invented the mRNA [vaccine] and has dedicated his life to vaccines.
Read: How mRNA technology could change the worldWherever he appears, Malone is billed as the inventor of mRNA vaccines.
Why is the self-described inventor of the mRNA vaccines working so hard to undermine them?
(Karikó is a senior vice president at BioNTech, which partnered with Pfizer to create the first COVID-19 vaccine to be authorized for use last year.)
Read: The mRNA vaccines are extraordinary, but Novavax is even betterHis concerns are personal, too.
0.048611111111111105
The Article is some-what positive
|
h2o/automl_binary_classification_product_backorders.ipynb | ###Markdown
H2O AutoML Binary Classification DemoThis is a [Jupyter](https://jupyter.org/) Notebook. When you execute code within the notebook, the results appear beneath the code. To execute a code chunk, place your cursor on the cell and press *Shift+Enter*. Start H2OImport the **h2o** Python module and `H2OAutoML` class and initialize a local H2O cluster.
###Code
import h2o
from h2o.automl import H2OAutoML
h2o.init(ip="localhost",max_mem_size_GB = 2)
###Output
_____no_output_____
###Markdown
Load DataFor the AutoML binary classification demo, we use a subset of the [Product Backorders](https://www.kaggle.com/tiredgeek/predict-bo-trial/data) dataset. The goal here is to predict whether or not a product will be put on backorder status, given a number of product metrics such as current inventory, transit time, demand forecasts and prior sales.
###Code
# Use local data file or download from GitHub
import os
docker_data_path = "/home/h2o/data/automl/product_backorders.csv"
if os.path.isfile(docker_data_path):
data_path = docker_data_path
else:
data_path = "https://github.com/h2oai/h2o-tutorials/raw/master/h2o-world-2017/automl/data/product_backorders.csv"
# Load data into H2O
df = h2o.import_file(data_path)
###Output
_____no_output_____
###Markdown
For classification, the response should be encoded as categorical (aka. "factor" or "enum"). Let's take a look.
###Code
df.describe()
###Output
_____no_output_____
###Markdown
We will notice that the response column, `"went_on_backorder"`, is already encoded as "enum", so there's nothing we need to do here. If it were encoded as a 0/1 "int", then we'd have to convert the column as follows: `df[y] = df[y].asfactor()`Next, let's identify the response & predictor columns by saving them as `x` and `y`. The `"sku"` column is a unique identifier so we'll want to remove that from the set of our predictors.
###Code
y = "went_on_backorder"
x = df.columns
x.remove(y)
x.remove("sku")
###Output
_____no_output_____
###Markdown
Run AutoML Run AutoML, stopping after 10 models. The `max_models` argument specifies the number of individual (or "base") models, and does not include the two ensemble models that are trained at the end.
###Code
aml = H2OAutoML(max_models = 10, seed = 1)
aml.train(x = x, y = y, training_frame = df)
###Output
_____no_output_____
###Markdown
*Note: If you see the following error, it means that you need to install the pandas module.*```H2OTypeError: Argument `python_obj` should be a None | list | tuple | dict | numpy.ndarray | pandas.DataFrame | scipy.sparse.issparse, got H2OTwoDimTable ``` LeaderboardNext, we will view the AutoML Leaderboard. Since we did not specify a `leaderboard_frame` in the `H2OAutoML.train()` method for scoring and ranking the models, the AutoML leaderboard uses cross-validation metrics to rank the models. A default performance metric for each machine learning task (binary classification, multiclass classification, regression) is specified internally and the leaderboard will be sorted by that metric. In the case of binary classification, the default ranking metric is Area Under the ROC Curve (AUC). In the future, the user will be able to specify any of the H2O metrics so that different metrics can be used to generate rankings on the leaderboard.The leader model is stored at `aml.leader` and the leaderboard is stored at `aml.leaderboard`.
###Code
lb = aml.leaderboard
###Output
_____no_output_____
###Markdown
Now we will view a snapshot of the top models. Here we should see the two Stacked Ensembles at or near the top of the leaderboard. Stacked Ensembles can almost always outperform a single model.
###Code
lb.head()
###Output
_____no_output_____
###Markdown
To view the entire leaderboard, specify the `rows` argument of the `head()` method as the total number of rows:
###Code
lb.head(rows=lb.nrows)
###Output
_____no_output_____
###Markdown
Ensemble ExplorationTo understand how the ensemble works, let's take a peek inside the Stacked Ensemble "All Models" model. The "All Models" ensemble is an ensemble of all of the individual models in the AutoML run. This is often the top performing model on the leaderboard.
###Code
# Get model ids for all models in the AutoML Leaderboard
model_ids = list(aml.leaderboard['model_id'].as_data_frame().iloc[:,0])
# Get the "All Models" Stacked Ensemble model
se = h2o.get_model([mid for mid in model_ids if "StackedEnsemble_AllModels" in mid][0])
# Get the Stacked Ensemble metalearner model
metalearner = h2o.get_model(se.metalearner()['name'])
###Output
_____no_output_____
###Markdown
Examine the variable importance of the metalearner (combiner) algorithm in the ensemble. This shows us how much each base learner is contributing to the ensemble. The AutoML Stacked Ensembles use the default metalearner algorithm (GLM with non-negative weights), so the variable importance of the metalearner is actually the standardized coefficient magnitudes of the GLM.
###Code
metalearner.coef_norm()
###Output
_____no_output_____
###Markdown
We can also plot the base learner contributions to the ensemble.
###Code
%matplotlib inline
metalearner.std_coef_plot()
###Output
_____no_output_____
###Markdown
Save Leader ModelThere are two ways to save the leader model -- binary format and MOJO format. If you're taking your leader model to production, then we'd suggest the MOJO format since it's optimized for production use.
###Code
h2o.save_model(aml.leader, path = "./product_backorders_model_bin")
aml.leader.download_mojo(path = "./")
###Output
_____no_output_____ |
EM/Probabilistic Graphical Models- EM Algorithm.ipynb | ###Markdown
Probabilistic Graphical Models —EM— **Author:** Mithuran GAJENDRAN & Hugo MALLET Imports
###Code
# Imports
import numpy as np
import matplotlib.pyplot as plt
from scipy.stats import chi2
from matplotlib.patches import Ellipse
from matplotlib.animation import FuncAnimation
###Output
_____no_output_____
###Markdown
Plot functions
###Code
# All plot functions are included here
def plot_ellipses(A, mu,col):
"""
Inputs
----------
A: Matrix
mu: means of the mixture model (array)
col: color of the ellipse
Outputs
-------
None, Display only
"""
N = 36
th = np.arange(0, 2 * np.pi + np.pi / N, np.pi / N)
X = np.array([np.cos(th), np.sin(th)])
Y = np.dot(A, X)
ln = plt.Line2D(mu[0] + Y[0,:], mu[1] + Y[1,:], c=col, linewidth=2)
return ln
def plot(x,mu, sigma, true=False):
"""
Inputs
----------
x: x distribution
mu: means of the mixture model (array)
sigma: the standard-deviation scalars (array)
true: plots either true or estimated Gaussians (Boolean)
Outputs
-------
None, Display only
"""
plt.figure(figsize=(15,8))
plt.rc('text', usetex=False)
if true:
plt.title("Contours of the true Gaussians")
else:
plt.title("Contours of the estimated Gaussians")
plt.grid()
ax=plt.gca()
for i in range(K):
A = np.linalg.cholesky(sigma[i])
x_plot = plt.plot(x[i][:,0],x[i][:,1],'.',color=color_list[i],label="Gaussienne n°%d"%i)
mean_plot = plt.plot(mu[i][0],mu[i][1],'^k',markersize=15)
ax.add_line(plot_ellipses(A,mu[i],"k"))
def plot_comparison(x,true_mu,true_sigma,mu,sigma):
"""
Inputs
----------
x: x distribution
true_mu: means of the mixture model (array)
true_sigma: the standard-deviation scalars (array)
mu: means of the mixture model (array)
sigma: the standard-deviation scalars (array)
Outputs
-------
None, Display only
"""
plt.figure(figsize=(15,8))
plt.title("Contours of the estimated vs true Gaussians")
plt.grid()
ax=plt.gca()
for i in range(K):
true_A = np.linalg.cholesky(true_sigma[i])
estimated_A = np.linalg.cholesky(sigma[i])
x_plot = plt.plot(x[i][:,0],x[i][:,1],'.',color=color_list[i])
true_mean_plot = plt.plot(true_mu[i][0],true_mu[i][1],'^k',mec="g",mfc=color_list[i], markersize=20)
estimated_mean_plot = plt.plot(mu[i][0],mu[i][1],'^',mec=color_list[i],mfc='black',markersize=15)
ax.add_line(plot_ellipses(true_A,true_mu[i],color_list[i]))
ax.add_line(plot_ellipses(estimated_A,mu[i],'k'))
plt.legend()
plt.show()
###Output
_____no_output_____
###Markdown
EM for GMMs The Expectation-Maximisation algorithm was proposed by Dempster in 1977. The first idea is to assume that the data is generated by a mixture model.For a particular observation $y$ we have$ p(y) = \sum_{g=1}^G \eta_g \ p(y|z=g) $The parameters to estimate are $\eta_g$ and $ \mu_g$, $\Sigma_g$ if we consider Gaussian distributions. For that the algorithm iterates on two steps:The **E-step**: - we assume that we know the Gaussian parameters and the prior - we plug the known parameters in $p(y)$, and with $l_{ig} = \eta_g \ p(y_i|z_i=g)$ we have:\begin{aligned}l_{ig} = \eta_g \times \frac 1 {\sqrt{2\pi}^d |\Sigma_g|^{-1}} \exp{\Big( -\frac{1}{2} (y_j-\mu_i)^T \Sigma^{-1}_{i} (y_j-\mu_i) \Big)}\end{aligned}The **M-step**:- we now figure out what those parameters should have been by updating them- $\eta_g = \frac{\sum_{i}l_{ig}}{n}$ is the mean of the probability of datapoints divided by their total number $n$.- $\mu_g = \frac{\sum_{i}l_{ig}*y_i}{\sum_{i}l_{ig}} $ is the weighted mean of the probability - $ \Sigma_g = \frac{\sum_{i}l_{ig}*(y_i-\mu_g)^T(y_i-\mu_g)}{\sum_{i}l_{ig}} $Those are just the calculation of the parameters over the soft correspondance $l_{ig}$ of datapoints to their gaussian. Question 1 
###Code
def log_sum_exp(v, axis=0):
"""
Inputs
----------
v: vector
Outputs
-------
v: vector with no NaN issues
"""
v_max = np.max(v)
return v_max + np.log(np.sum(np.exp(v - v_max)))
def log_det(A):
diag = np.diag(np.linalg.cholesky(A))
return 2 * np.sum(np.log(diag))
def comp_li(pi_i,x,mu_i,sigma_i):
"""
Inputs
----------
pi_i: eta_i, data point probability i in a group
x : x datapoints
mu_i: mean i of the mixture model (array)
sigma_i: i-array of the standard-deviation
Outputs
-------
li: the probability for each datapointsto be part of cluster number i
"""
d=len(mu_i)
li= np.log(pi_i) - d *np.log(2 *np.pi) /2 - log_det(sigma_i)/2 - (x-mu_i)[email protected](sigma_i)@(x-mu_i)/2
return li
def comp_gamma_i(pi,x,mu,sigma,i):
"""
Inputs
----------
pi: mean of data point probability
x : x datapoints
mu: means of the mixture model (array)
sigma: the standard-deviation scalars (array)
i: index
Outputs
-------
gamma_i: posterior i
"""
K = len(pi)
v = np.zeros(K)
for k in range(K):
v[k] = comp_li(pi[k],x, mu[k], sigma[k])
return np.exp(v[i] - log_sum_exp(v))
###Output
_____no_output_____
###Markdown
 Question 3  1_
###Code
#Parameters
N=1000
K=3
true_pi= np.array([0.1,0.2,0.5])
true_mu=np.array([[0,0],[1,2],[2,0]])
true_sigma= np.array([[[1.00, -0.25], [-0.25, 0.50]],[[0.50, 0.25],[0.25, 0.50]],[[0.50, -0.25],[ -0.25, 1]]])
#Nb of values -n- in each distributions
list_N=np.random.multinomial(N, true_pi)
#list_x is a list of lists containing each distributions
list_x=[]
for i in range(K):
list_x.append(np.random.multivariate_normal(true_mu[i], true_sigma[i], list_N[i]))
#X is the jointure of all the lists in x_list in one list
X=np.vstack(list_x)
#First simple plot to see data
plt.figure(figsize=(15,8))
plt.grid()
color_list=["deepskyblue","deeppink","darkorange","forestgreen","blueviolet"] #funky color, because we like it
for i in range(K):
x_plot = plt.plot(list_x[i][:,0],list_x[i][:,1],'.',color=color_list[i],label="Gaussienne n°%d"%i)
mean_plot = plt.plot(true_mu[i,0],true_mu[i,1],'^k',markersize=15,mec = color_list[i])
plt.legend([(mean_plot),("Mean of each distribution",)])
plt.title("Plot of a multivariate normal distribution", fontsize="20")
plt.show()
###Output
_____no_output_____
###Markdown
2_
###Code
## ALGORITHM EM
def log_likelihood(x,gamma, pi, mu, sigma):
"""
Inputs
----------
x: y dataset
gamma : matrix
mu: means of the mixture model (array)
sigma: array of the standard-deviation scalars
Outputs
-------
log_like: likelihood
"""
log_like = 0
for n in range(N):
for k in range(K):
log_like += gamma[n,k] * comp_li(pi[k],x[n], mu[k], sigma[k])
return log_like
def EM(x,number_iteration, pi, mu, sigma, likelihood=False):
"""
Inputs
----------
x:y dataset
pi : eta a priori probabilities
mu: means of the mixture model (array)
sigma: array of the standard-deviation scalars
Outputs
-------
pi: eta a priori probabilities
mu: means of the mixture model (array)
sigma: array of the standard-deviation scalars
likelihood_array: likelihood array
time_array: time array
"""
likelihood_array = []
plot_each=number_iteration//3
for i in range(number_iteration):
# E step
for n in range(N):
for k in range(K):
gamma[n,k] = comp_gamma_i(pi,x[n],mu,sigma,k)
# Likelihood
if likelihood:
likelihood_array.append(log_likelihood(x,gamma, pi, mu, sigma))
# M step
for k in range(K):
mu[k] = np.zeros(len(mu[0]))
for n in range(N):
mu[k] += gamma[n,k] * x[n]
mu[k] /= np.sum(gamma[:,k])
sigma = np.zeros((K, 2, 2))
for k in range(K):
for n in range(N):
A = np.reshape(x[n] - mu[k], (2,1))
sigma[k] += gamma[n,k] * np.dot(A, A.T)
sigma[k] /= np.sum(gamma[:,k])
# PI
for k in range(K):
pi[k] = np.average(gamma[:,k])
if i%plot_each==0:
plot(list_x,mu,sigma)
return pi, mu, sigma, likelihood_array
###Output
_____no_output_____
###Markdown
 2_a
###Code
#Initial parameters RANDOM
pi = np.random.rand(K)
pi = pi / np.sum(pi)
mu = []
sigma = []
for i in range(K):
mu.append(np.random.randint(-1,5,2)) # for instance
A = np.random.rand(2,2)
sigma.append(list(A.dot(A.T)))
gamma = np.zeros((N, K))
pi, mu, sigma, likelihood_array = EM(X,100, pi, mu, sigma,likelihood=True)
plot(list_x,true_mu,true_sigma,True)
###Output
_____no_output_____
###Markdown
2_b
###Code
plt.figure(figsize=(15,8))
plt.title("Log-Likelihood vs Iterations", fontsize=15)
plt.plot(likelihood_array)
plt.show()
print("In black: estimated gaussians\nIn color: real gaussians")
plot_comparison(list_x,true_mu,true_sigma,mu, sigma)
###Output
No handles with labels found to put in legend.
|
LAB 9.ipynb | ###Markdown
Collect Tweets into MongoDB Install Python librariesYou may need to restart your Jupyter Notebook instance after installed those libraries.
###Code
!pip install pymongo
!pip install pymongo[srv]
!pip install dnspython
!pip install tweepy
!pip install twitter
###Output
Collecting twitter
Downloading twitter-1.19.3-py2.py3-none-any.whl (50 kB)
[K |████████████████████████████████| 50 kB 6.8 MB/s eta 0:00:01
[?25hInstalling collected packages: twitter
Successfully installed twitter-1.19.3
[33mWARNING: You are using pip version 21.2.4; however, version 21.3.1 is available.
You should consider upgrading via the '/home/ec2-user/anaconda3/envs/python3/bin/python -m pip install --upgrade pip' command.[0m
###Markdown
Import Python libraries
###Code
import pymongo
from pymongo import MongoClient
import json
import tweepy
import twitter
from pprint import pprint
import configparser
import pandas as pd
###Output
_____no_output_____
###Markdown
Load the Authorization Info Save database connection info and API Keys in a config.ini file and use the configparse to load the authorization info.
###Code
config = configparser.ConfigParser()
config.read('config.ini')
CONSUMER_KEY = config['mytwitter']['api_key']
CONSUMER_SECRET = config['mytwitter']['api_secrete']
OAUTH_TOKEN = config['mytwitter']['access_token']
OATH_TOKEN_SECRET = config['mytwitter']['access_secrete']
mongod_connect = config['mymongo']['connection']
###Output
_____no_output_____
###Markdown
Connect to the MongoDB Cluster
###Code
client = MongoClient(mongod_connect)
db = client.gp6 # use or create a database named demo
tweet_collection = db.lab9 #use or create a collection named tweet_collection
tweet_collection.create_index([("id", pymongo.ASCENDING)],unique = True) # make sure the collected tweets are unique
###Output
_____no_output_____
###Markdown
Use the Streaming API to Collect Tweets Authorize the Stream API
###Code
stream_auth = tweepy.OAuthHandler(CONSUMER_KEY, CONSUMER_SECRET)
stream_auth.set_access_token(OAUTH_TOKEN, OATH_TOKEN_SECRET)
strem_api = tweepy.API(stream_auth)
###Output
_____no_output_____
###Markdown
Define the query for the Stream API
###Code
track = ['covid19'] # define the keywords, tweets contain election
locations = [-78.9326449,38.4150904,-78.8816972,38.4450731] #defin the location, in Harrisonburg, VA
###Output
_____no_output_____
###Markdown
The collected tweets will contain 'election' OR are located in Harrisonburg, VA
###Code
class MyStreamListener(tweepy.StreamListener):
def on_status(self, status):
print (status.id_str)
try:
tweet_collection.insert_one(status._json)
except:
pass
def on_error(self, status_code):
if status_code == 420:
#returning False in on_data disconnects the stream
return False
myStreamListener = MyStreamListener()
myStream = tweepy.Stream(auth = strem_api.auth, listener=myStreamListener)
myStream.filter(track=track)# (locations = locations) #Use either track or locations
###Output
_____no_output_____
###Markdown
Use the REST API to Collect Tweets Authorize the REST API
###Code
rest_auth = twitter.oauth.OAuth(OAUTH_TOKEN,OATH_TOKEN_SECRET,CONSUMER_KEY,CONSUMER_SECRET)
rest_api = twitter.Twitter(auth=rest_auth)
###Output
_____no_output_____
###Markdown
Define the query for the REST API
###Code
count = 100 #number of returned tweets, default and max is 100
geocode = "38.4392897,-78.9412224,50mi" # defin the location, in Harrisonburg, VA
q = "covid" #define the keywords, tweets contain election
###Output
_____no_output_____
###Markdown
The collected tweets will contain 'election' AND are located in Harrisonburg, VA
###Code
search_results = rest_api.search.tweets( count=count,q=q, geocode=geocode) #you can use both q and geocode
statuses = search_results["statuses"]
since_id_new = statuses[-1]['id']
for statuse in statuses:
try:
tweet_collection.insert_one(statuse)
pprint(statuse['created_at'])# print the date of the collected tweets
except:
pass
###Output
'Fri Dec 10 19:16:58 +0000 2021'
'Fri Dec 10 19:10:19 +0000 2021'
'Fri Dec 10 19:09:25 +0000 2021'
'Fri Dec 10 19:08:55 +0000 2021'
'Fri Dec 10 19:07:03 +0000 2021'
'Fri Dec 10 19:00:22 +0000 2021'
'Fri Dec 10 19:00:01 +0000 2021'
'Fri Dec 10 18:57:48 +0000 2021'
'Fri Dec 10 18:55:41 +0000 2021'
'Fri Dec 10 18:50:52 +0000 2021'
'Fri Dec 10 18:41:49 +0000 2021'
'Fri Dec 10 18:41:21 +0000 2021'
'Fri Dec 10 18:39:52 +0000 2021'
'Fri Dec 10 18:35:42 +0000 2021'
'Fri Dec 10 18:30:00 +0000 2021'
'Fri Dec 10 18:09:20 +0000 2021'
'Fri Dec 10 18:08:22 +0000 2021'
'Fri Dec 10 18:06:10 +0000 2021'
'Fri Dec 10 18:05:32 +0000 2021'
'Fri Dec 10 17:48:47 +0000 2021'
'Fri Dec 10 17:45:27 +0000 2021'
'Fri Dec 10 17:39:31 +0000 2021'
'Fri Dec 10 17:35:07 +0000 2021'
'Fri Dec 10 17:34:11 +0000 2021'
'Fri Dec 10 17:29:28 +0000 2021'
'Fri Dec 10 17:21:48 +0000 2021'
'Fri Dec 10 17:19:52 +0000 2021'
'Fri Dec 10 17:16:06 +0000 2021'
'Fri Dec 10 17:07:03 +0000 2021'
'Fri Dec 10 17:02:53 +0000 2021'
'Fri Dec 10 16:49:08 +0000 2021'
'Fri Dec 10 16:47:29 +0000 2021'
'Fri Dec 10 16:42:36 +0000 2021'
'Fri Dec 10 16:39:10 +0000 2021'
'Fri Dec 10 16:31:51 +0000 2021'
'Fri Dec 10 16:26:06 +0000 2021'
'Fri Dec 10 16:25:48 +0000 2021'
'Fri Dec 10 16:22:35 +0000 2021'
'Fri Dec 10 16:22:18 +0000 2021'
'Fri Dec 10 16:20:56 +0000 2021'
'Fri Dec 10 16:17:53 +0000 2021'
'Fri Dec 10 16:01:38 +0000 2021'
'Fri Dec 10 15:52:43 +0000 2021'
'Fri Dec 10 15:45:15 +0000 2021'
'Fri Dec 10 15:44:17 +0000 2021'
'Fri Dec 10 15:38:21 +0000 2021'
'Fri Dec 10 15:35:55 +0000 2021'
'Fri Dec 10 15:21:58 +0000 2021'
'Fri Dec 10 15:21:30 +0000 2021'
'Fri Dec 10 15:08:55 +0000 2021'
'Fri Dec 10 15:08:29 +0000 2021'
'Fri Dec 10 15:04:45 +0000 2021'
'Fri Dec 10 14:58:07 +0000 2021'
'Fri Dec 10 14:55:21 +0000 2021'
'Fri Dec 10 14:55:09 +0000 2021'
'Fri Dec 10 14:53:32 +0000 2021'
'Fri Dec 10 14:52:14 +0000 2021'
'Fri Dec 10 14:51:29 +0000 2021'
'Fri Dec 10 14:51:19 +0000 2021'
'Fri Dec 10 14:42:45 +0000 2021'
'Fri Dec 10 14:40:11 +0000 2021'
'Fri Dec 10 14:36:02 +0000 2021'
'Fri Dec 10 14:17:47 +0000 2021'
'Fri Dec 10 14:15:57 +0000 2021'
'Fri Dec 10 14:15:52 +0000 2021'
'Fri Dec 10 14:15:05 +0000 2021'
'Fri Dec 10 14:10:48 +0000 2021'
'Fri Dec 10 14:07:28 +0000 2021'
'Fri Dec 10 14:02:39 +0000 2021'
'Fri Dec 10 13:57:26 +0000 2021'
'Fri Dec 10 13:52:00 +0000 2021'
'Fri Dec 10 13:42:36 +0000 2021'
'Fri Dec 10 13:39:05 +0000 2021'
'Fri Dec 10 13:37:24 +0000 2021'
'Fri Dec 10 13:25:44 +0000 2021'
'Fri Dec 10 13:18:04 +0000 2021'
'Fri Dec 10 13:18:00 +0000 2021'
'Fri Dec 10 13:05:00 +0000 2021'
'Fri Dec 10 12:48:57 +0000 2021'
'Fri Dec 10 12:43:41 +0000 2021'
'Fri Dec 10 12:33:43 +0000 2021'
'Fri Dec 10 12:30:33 +0000 2021'
'Fri Dec 10 12:30:11 +0000 2021'
'Fri Dec 10 12:25:38 +0000 2021'
'Fri Dec 10 12:20:42 +0000 2021'
'Fri Dec 10 12:16:52 +0000 2021'
'Fri Dec 10 12:10:57 +0000 2021'
'Fri Dec 10 12:09:36 +0000 2021'
'Fri Dec 10 12:08:38 +0000 2021'
'Fri Dec 10 12:08:14 +0000 2021'
'Fri Dec 10 12:02:36 +0000 2021'
'Fri Dec 10 12:01:03 +0000 2021'
'Fri Dec 10 12:00:06 +0000 2021'
'Fri Dec 10 12:00:03 +0000 2021'
'Fri Dec 10 11:59:29 +0000 2021'
'Fri Dec 10 11:53:57 +0000 2021'
'Fri Dec 10 11:40:28 +0000 2021'
'Fri Dec 10 11:32:42 +0000 2021'
'Fri Dec 10 11:25:45 +0000 2021'
'Fri Dec 10 11:18:40 +0000 2021'
###Markdown
Continue fetching early tweets with the same query. YOU WILL REACH YOUR RATE LIMIT VERY FAST
###Code
since_id_old = 0
while(since_id_new != since_id_old):
since_id_old = since_id_new
search_results = rest_api.search.tweets( count=count,q=q,
geocode=geocode, max_id= since_id_new)
statuses = search_results["statuses"]
since_id_new = statuses[-1]['id']
for statuse in statuses:
try:
tweet_collection.insert_one(statuse)
pprint(statuse['created_at']) # print the date of the collected tweets
except:
pass
###Output
'Fri Dec 10 11:13:32 +0000 2021'
'Fri Dec 10 11:09:24 +0000 2021'
'Fri Dec 10 10:58:09 +0000 2021'
'Fri Dec 10 10:24:58 +0000 2021'
'Fri Dec 10 09:12:29 +0000 2021'
'Fri Dec 10 09:01:17 +0000 2021'
'Fri Dec 10 08:58:28 +0000 2021'
'Fri Dec 10 08:33:18 +0000 2021'
'Fri Dec 10 08:33:17 +0000 2021'
'Fri Dec 10 08:28:27 +0000 2021'
'Fri Dec 10 08:26:47 +0000 2021'
'Fri Dec 10 08:24:26 +0000 2021'
'Fri Dec 10 08:21:04 +0000 2021'
'Fri Dec 10 07:47:17 +0000 2021'
'Fri Dec 10 07:45:38 +0000 2021'
'Fri Dec 10 07:14:26 +0000 2021'
'Fri Dec 10 06:05:36 +0000 2021'
'Fri Dec 10 05:54:50 +0000 2021'
'Fri Dec 10 05:45:59 +0000 2021'
'Fri Dec 10 05:25:49 +0000 2021'
'Fri Dec 10 05:00:42 +0000 2021'
'Fri Dec 10 04:33:29 +0000 2021'
'Fri Dec 10 04:31:28 +0000 2021'
'Fri Dec 10 04:09:14 +0000 2021'
'Fri Dec 10 04:07:37 +0000 2021'
'Fri Dec 10 04:04:55 +0000 2021'
'Fri Dec 10 04:02:46 +0000 2021'
'Fri Dec 10 03:51:26 +0000 2021'
'Fri Dec 10 03:33:07 +0000 2021'
'Fri Dec 10 03:27:28 +0000 2021'
'Fri Dec 10 03:11:35 +0000 2021'
'Fri Dec 10 03:02:18 +0000 2021'
'Fri Dec 10 02:55:04 +0000 2021'
'Fri Dec 10 02:29:25 +0000 2021'
'Fri Dec 10 02:17:53 +0000 2021'
'Fri Dec 10 02:15:00 +0000 2021'
'Fri Dec 10 02:11:49 +0000 2021'
'Fri Dec 10 02:05:57 +0000 2021'
'Fri Dec 10 02:05:48 +0000 2021'
'Fri Dec 10 01:49:14 +0000 2021'
'Fri Dec 10 01:48:08 +0000 2021'
'Fri Dec 10 01:45:06 +0000 2021'
'Fri Dec 10 01:36:30 +0000 2021'
'Fri Dec 10 01:35:05 +0000 2021'
'Fri Dec 10 01:20:46 +0000 2021'
'Fri Dec 10 01:10:16 +0000 2021'
'Fri Dec 10 00:59:39 +0000 2021'
'Fri Dec 10 00:42:38 +0000 2021'
'Fri Dec 10 00:15:04 +0000 2021'
'Fri Dec 10 00:13:37 +0000 2021'
'Fri Dec 10 00:11:54 +0000 2021'
'Fri Dec 10 00:02:03 +0000 2021'
'Thu Dec 09 23:50:39 +0000 2021'
'Thu Dec 09 23:43:37 +0000 2021'
'Thu Dec 09 23:40:21 +0000 2021'
'Thu Dec 09 23:15:02 +0000 2021'
'Thu Dec 09 23:06:24 +0000 2021'
'Thu Dec 09 23:04:50 +0000 2021'
'Thu Dec 09 22:48:54 +0000 2021'
'Thu Dec 09 22:48:48 +0000 2021'
'Thu Dec 09 22:41:33 +0000 2021'
'Thu Dec 09 22:34:07 +0000 2021'
'Thu Dec 09 22:33:00 +0000 2021'
'Thu Dec 09 22:26:44 +0000 2021'
'Thu Dec 09 22:13:14 +0000 2021'
'Thu Dec 09 21:48:44 +0000 2021'
'Thu Dec 09 21:47:12 +0000 2021'
'Thu Dec 09 21:38:20 +0000 2021'
'Thu Dec 09 21:38:19 +0000 2021'
'Thu Dec 09 21:38:19 +0000 2021'
'Thu Dec 09 21:33:51 +0000 2021'
'Thu Dec 09 21:30:42 +0000 2021'
'Thu Dec 09 21:30:06 +0000 2021'
'Thu Dec 09 21:28:43 +0000 2021'
'Thu Dec 09 21:20:58 +0000 2021'
'Thu Dec 09 21:19:23 +0000 2021'
'Thu Dec 09 21:07:59 +0000 2021'
'Thu Dec 09 21:05:34 +0000 2021'
'Thu Dec 09 21:03:24 +0000 2021'
'Thu Dec 09 20:40:47 +0000 2021'
'Thu Dec 09 20:38:53 +0000 2021'
'Thu Dec 09 20:33:29 +0000 2021'
'Thu Dec 09 20:30:10 +0000 2021'
'Thu Dec 09 20:19:51 +0000 2021'
'Thu Dec 09 20:16:46 +0000 2021'
'Thu Dec 09 20:13:56 +0000 2021'
'Thu Dec 09 20:12:42 +0000 2021'
'Thu Dec 09 20:07:12 +0000 2021'
'Thu Dec 09 20:00:11 +0000 2021'
'Thu Dec 09 19:45:39 +0000 2021'
'Thu Dec 09 19:44:35 +0000 2021'
'Thu Dec 09 19:30:10 +0000 2021'
'Thu Dec 09 19:30:00 +0000 2021'
'Thu Dec 09 19:21:29 +0000 2021'
'Thu Dec 09 19:19:29 +0000 2021'
'Thu Dec 09 19:07:40 +0000 2021'
'Thu Dec 09 19:05:20 +0000 2021'
'Thu Dec 09 18:47:30 +0000 2021'
'Thu Dec 09 18:46:45 +0000 2021'
'Thu Dec 09 18:46:43 +0000 2021'
'Thu Dec 09 18:23:44 +0000 2021'
'Thu Dec 09 18:15:37 +0000 2021'
'Thu Dec 09 17:57:01 +0000 2021'
'Thu Dec 09 17:46:31 +0000 2021'
'Thu Dec 09 17:42:40 +0000 2021'
'Thu Dec 09 17:37:25 +0000 2021'
'Thu Dec 09 17:37:08 +0000 2021'
'Thu Dec 09 17:33:04 +0000 2021'
'Thu Dec 09 17:33:03 +0000 2021'
'Thu Dec 09 17:30:06 +0000 2021'
'Thu Dec 09 17:20:07 +0000 2021'
'Thu Dec 09 17:02:09 +0000 2021'
'Thu Dec 09 16:58:07 +0000 2021'
'Thu Dec 09 16:58:04 +0000 2021'
'Thu Dec 09 16:44:15 +0000 2021'
'Thu Dec 09 16:43:23 +0000 2021'
'Thu Dec 09 16:42:04 +0000 2021'
'Thu Dec 09 16:40:23 +0000 2021'
'Thu Dec 09 16:38:40 +0000 2021'
'Thu Dec 09 16:36:08 +0000 2021'
'Thu Dec 09 16:34:00 +0000 2021'
'Thu Dec 09 16:28:08 +0000 2021'
'Thu Dec 09 16:18:00 +0000 2021'
'Thu Dec 09 16:16:11 +0000 2021'
'Thu Dec 09 16:14:29 +0000 2021'
'Thu Dec 09 16:11:23 +0000 2021'
'Thu Dec 09 16:10:30 +0000 2021'
'Thu Dec 09 15:57:09 +0000 2021'
'Thu Dec 09 15:52:40 +0000 2021'
'Thu Dec 09 15:51:18 +0000 2021'
'Thu Dec 09 15:45:08 +0000 2021'
'Thu Dec 09 15:34:45 +0000 2021'
'Thu Dec 09 15:31:06 +0000 2021'
'Thu Dec 09 15:26:20 +0000 2021'
'Thu Dec 09 15:21:48 +0000 2021'
'Thu Dec 09 15:17:41 +0000 2021'
'Thu Dec 09 15:17:33 +0000 2021'
'Thu Dec 09 15:14:15 +0000 2021'
'Thu Dec 09 15:03:43 +0000 2021'
'Thu Dec 09 15:03:11 +0000 2021'
'Thu Dec 09 15:03:04 +0000 2021'
'Thu Dec 09 15:00:15 +0000 2021'
'Thu Dec 09 14:54:59 +0000 2021'
'Thu Dec 09 14:54:41 +0000 2021'
'Thu Dec 09 14:48:59 +0000 2021'
'Thu Dec 09 14:43:27 +0000 2021'
'Thu Dec 09 14:40:05 +0000 2021'
'Thu Dec 09 14:38:23 +0000 2021'
'Thu Dec 09 14:34:36 +0000 2021'
'Thu Dec 09 14:30:03 +0000 2021'
'Thu Dec 09 14:24:32 +0000 2021'
'Thu Dec 09 14:18:20 +0000 2021'
'Thu Dec 09 14:15:46 +0000 2021'
'Thu Dec 09 14:15:05 +0000 2021'
'Thu Dec 09 14:06:40 +0000 2021'
'Thu Dec 09 14:04:49 +0000 2021'
'Thu Dec 09 13:57:02 +0000 2021'
'Thu Dec 09 13:50:43 +0000 2021'
'Thu Dec 09 13:48:42 +0000 2021'
'Thu Dec 09 13:44:56 +0000 2021'
'Thu Dec 09 13:40:11 +0000 2021'
'Thu Dec 09 13:26:27 +0000 2021'
'Thu Dec 09 13:20:50 +0000 2021'
'Thu Dec 09 13:20:04 +0000 2021'
'Thu Dec 09 13:18:05 +0000 2021'
'Thu Dec 09 13:16:45 +0000 2021'
'Thu Dec 09 13:15:38 +0000 2021'
'Thu Dec 09 13:14:30 +0000 2021'
'Thu Dec 09 13:14:19 +0000 2021'
'Thu Dec 09 13:13:52 +0000 2021'
'Thu Dec 09 13:12:10 +0000 2021'
'Thu Dec 09 12:57:16 +0000 2021'
'Thu Dec 09 12:47:44 +0000 2021'
'Thu Dec 09 12:44:49 +0000 2021'
'Thu Dec 09 12:41:56 +0000 2021'
'Thu Dec 09 12:37:16 +0000 2021'
'Thu Dec 09 12:33:34 +0000 2021'
'Thu Dec 09 12:24:55 +0000 2021'
'Thu Dec 09 12:22:28 +0000 2021'
'Thu Dec 09 12:22:04 +0000 2021'
'Thu Dec 09 12:15:41 +0000 2021'
'Thu Dec 09 12:13:39 +0000 2021'
'Thu Dec 09 12:11:36 +0000 2021'
'Thu Dec 09 12:10:14 +0000 2021'
'Thu Dec 09 12:01:43 +0000 2021'
'Thu Dec 09 12:00:02 +0000 2021'
'Thu Dec 09 11:54:09 +0000 2021'
'Thu Dec 09 11:40:38 +0000 2021'
'Thu Dec 09 11:39:37 +0000 2021'
'Thu Dec 09 11:27:42 +0000 2021'
'Thu Dec 09 11:26:41 +0000 2021'
'Thu Dec 09 11:09:53 +0000 2021'
'Thu Dec 09 11:05:41 +0000 2021'
'Thu Dec 09 10:56:52 +0000 2021'
'Thu Dec 09 10:52:26 +0000 2021'
'Thu Dec 09 10:46:43 +0000 2021'
'Thu Dec 09 10:45:36 +0000 2021'
'Thu Dec 09 10:45:02 +0000 2021'
'Thu Dec 09 10:31:39 +0000 2021'
'Thu Dec 09 10:11:44 +0000 2021'
'Thu Dec 09 09:58:10 +0000 2021'
'Thu Dec 09 09:45:10 +0000 2021'
'Thu Dec 09 09:34:49 +0000 2021'
'Thu Dec 09 09:07:44 +0000 2021'
'Thu Dec 09 08:55:23 +0000 2021'
'Thu Dec 09 08:06:29 +0000 2021'
'Thu Dec 09 08:02:48 +0000 2021'
'Thu Dec 09 07:46:55 +0000 2021'
'Thu Dec 09 07:27:22 +0000 2021'
'Thu Dec 09 06:53:33 +0000 2021'
'Thu Dec 09 06:29:00 +0000 2021'
'Thu Dec 09 06:25:57 +0000 2021'
'Thu Dec 09 06:18:22 +0000 2021'
'Thu Dec 09 04:19:19 +0000 2021'
'Thu Dec 09 03:55:51 +0000 2021'
'Thu Dec 09 03:29:00 +0000 2021'
'Thu Dec 09 03:23:32 +0000 2021'
'Thu Dec 09 03:20:36 +0000 2021'
'Thu Dec 09 03:18:55 +0000 2021'
'Thu Dec 09 02:59:34 +0000 2021'
'Thu Dec 09 02:54:45 +0000 2021'
'Thu Dec 09 02:49:48 +0000 2021'
'Thu Dec 09 02:43:45 +0000 2021'
'Thu Dec 09 02:27:09 +0000 2021'
'Thu Dec 09 02:20:47 +0000 2021'
'Thu Dec 09 02:05:02 +0000 2021'
'Thu Dec 09 02:01:23 +0000 2021'
'Thu Dec 09 02:00:10 +0000 2021'
'Thu Dec 09 01:47:11 +0000 2021'
'Thu Dec 09 01:41:05 +0000 2021'
'Thu Dec 09 01:39:06 +0000 2021'
'Thu Dec 09 01:27:09 +0000 2021'
'Thu Dec 09 01:07:03 +0000 2021'
'Thu Dec 09 01:06:06 +0000 2021'
'Thu Dec 09 00:55:51 +0000 2021'
'Thu Dec 09 00:51:37 +0000 2021'
'Thu Dec 09 00:47:05 +0000 2021'
'Thu Dec 09 00:41:16 +0000 2021'
'Thu Dec 09 00:08:13 +0000 2021'
'Thu Dec 09 00:06:14 +0000 2021'
'Wed Dec 08 23:42:34 +0000 2021'
'Wed Dec 08 23:41:18 +0000 2021'
'Wed Dec 08 23:41:00 +0000 2021'
'Wed Dec 08 23:18:17 +0000 2021'
'Wed Dec 08 23:10:25 +0000 2021'
'Wed Dec 08 22:57:51 +0000 2021'
'Wed Dec 08 22:51:03 +0000 2021'
'Wed Dec 08 22:44:56 +0000 2021'
'Wed Dec 08 22:43:41 +0000 2021'
'Wed Dec 08 22:43:09 +0000 2021'
'Wed Dec 08 22:42:06 +0000 2021'
###Markdown
View the Collected Tweets Print the number of tweets and unique twitter users
###Code
print(tweet_collection.estimated_document_count())# number of tweets collected
user_cursor = tweet_collection.distinct("user.id")
print (len(user_cursor)) # number of unique Twitter users
###Output
1833
821
###Markdown
Create a text index and print the Tweets containing specific keywords.
###Code
tweet_collection.create_index([("text", pymongo.TEXT)], name='text_index', default_language='english') # create a text index
###Output
_____no_output_____
###Markdown
Create a cursor to query tweets with the created index
###Code
tweet_cursor = tweet_collection.find({"$text": {"$search": "vote"}}) # return tweets contain vote
###Output
_____no_output_____
###Markdown
Use pprint to display tweets
###Code
for document in tweet_cursor[0:10]: # display the first 10 tweets from the query
try:
print ('----')
# pprint (document) # use pprint to print the entire tweet document
print ('name:', document["user"]["name"]) # user name
print ('text:', document["text"]) # tweets
except:
print ("***error in encoding")
pass
tweet_cursor = tweet_collection.find({"$text": {"$search": "vote"}}) # return tweets contain vote
###Output
_____no_output_____
###Markdown
Use pandas to display tweets
###Code
tweet_df = pd.DataFrame(list(tweet_cursor ))
tweet_df[:10] #display the first 10 tweets
tweet_df["favorite_count"].hist() # create a histogram show the favorite count
###Output
_____no_output_____ |
notebooks/Jupyter_Magic.ipynb | ###Markdown
Jupyter Magic allows one notebook to have different programing languages. Everything in the following cell will be executed in bash This is very conventiant because Jupyter can now serve as a GUI text editor. Useful if you don't like using VIM, VI, or Nano in traditional high performance computing environments
###Code
%%bash
echo Test
pwd
cd ~
pwd
###Output
Test
/home/jovyan/cmip6_tutorial/notebooks
/home/jovyan
###Markdown
Now we are back to python
###Code
import numpy as np
test = 2
print(2)
###Output
2
###Markdown
And now we are in Latex
###Code
%%latex
\[
\hat{H}\Psi = E\Psi
\]
###Output
_____no_output_____
###Markdown
Pass variables from python to bash
###Code
# Python code
pythonvar = "This python variable is now passed to bash!"
%%bash -s "$pythonvar"
echo "$1"
###Output
This python variable is now passed to bash!
###Markdown
Now lets pass bash variables to python Some things are best computed on the command line, and visualized in python
###Code
bash_command = "pwd" # python
a = !echo pwd #python and bash
a #python
###Output
_____no_output_____ |
Week 3/Module 3 Graded Assignment.ipynb | ###Markdown
Module 3 AssignmentYour objective in this assignment is to implement a tennis ball detector using a pre-trained image classification network from GluonCV. We'll step through the pipeline, from loading and transforming an input image, to loading and using a pre-trained model. Since we're only interested in detecting tennis balls, this is a binary classification problem, which is slightly different to the multi-class classification setup we've seen so far. 0) SetupWe start with some initial setup: importing packages and setting the path to the data.
###Code
import mxnet as mx
import gluoncv as gcv
import matplotlib.pyplot as plt
import numpy as np
import os
from pathlib import Path
M3_DATA = Path(os.getenv('DATA_DIR', '../../data'), 'module_3')
M3_IMAGES = Path(M3_DATA, 'images')
M3_MODELS = Path(M3_DATA, 'models')
###Output
_____no_output_____
###Markdown
1) Loading an imageYour first task is to implement a function that loads an image from disk given a filepath.It should return an 8-bit image array, that's in MXNet's NDArray format and in HWC layout (i.e. height, width then channel).
###Code
def load_image(filepath):
"""
Should load image from disk.
:param filepath: relative or absolute filepath to RGB image file in JPG format.
:type filepath: str
:return: an array with pixel intensities (in HWC layout).
:rtype: mx.nd.NDArray
"""
return mx.image.imread(filepath)
test_filepath = Path(M3_IMAGES, 'ben-hershey-VEW78A1YZ6I-unsplash.jpg')
test_output = load_image(test_filepath)
assert test_output.shape[2] == 3 # RGB
assert test_output.dtype == np.uint8 # 0 - 255
assert isinstance(test_output, mx.nd.NDArray) # MXNet NDArray, not NumPy Array.
###Output
_____no_output_____
###Markdown
2) Transforming an imageUp next, you should transform the image so it can be used as input to the pre-trained network.Since we're going to use an ImageNet pre-trained network, we need to follow the same steps used for ImageNet pre-training.See the docstring for more details, but don't forget that GluonCV contains a number of utilities and helper functions to make your life easier! Check out the preset transforms.
###Code
from mxnet.gluon.data.vision import transforms
def transform_image(array):
"""
Should transform image by:
1) Resizing the shortest dimension to 224. e.g (448, 1792) -> (224, 896).
2) Cropping to a center square of dimension (224, 224).
3) Converting the image from HWC layout to CHW layout.
4) Normalizing the image using ImageNet statistics (i.e. per colour channel mean and variance).
5) Creating a batch of 1 image.
:param filepath: array (in HWC layout).
:type filepath: mx.nd.NDArray
:return: a batch of a single transformed images (in NCHW layout)
:rtype: mx.nd.NDArray
"""
train_trans = transforms.Compose([
transforms.Resize(224, keep_ratio=True),
transforms.CenterCrop((224,224)),
transforms.ToTensor(),
transforms.Normalize([.485, .456, .606], [.229, .224, .225])
])
return (train_trans(array).expand_dims(0))
transformed_test_output = transform_image(test_output)
assert transformed_test_output.shape == (1, 3, 224, 224)
assert transformed_test_output.dtype == np.float32
###Output
_____no_output_____
###Markdown
3) Loading a modelWith the image loaded and transformed, you now need to load a pre-trained classification model.Choose a MobileNet 1.0 image classification model that's been pre-trained on ImageNet.**CAUTION!**: Although the notebook interface has internet connectivity, the **autograders are not permitted to access the internet**. We have already downloaded the correct models and data for you to use so you don't need access to the internet. However, you do need to specify the correct path to the models when loading a model from the Gluon CV Model Zoo using `get_model` or otherwise. Set the `root` parameter to `M3_MODELS`. As an example, you should have something similar to `gcv.model_zoo.get_model(..., root=M3_MODELS)`. Usually, in the real world, you have internet access, so setting the `root` parameter isn't required (and it's set to `~/.mxnet` by default).
###Code
def load_pretrained_classification_network():
"""
Loads a MobileNet 1.0 network that's been pre-trained on ImageNet.
:return: a pre-trained network
:rtype: mx.gluon.Block
"""
return gcv.model_zoo.mobilenet1_0( root=M3_MODELS, pretrained=True )
network = load_pretrained_classification_network()
assert isinstance(network, mx.gluon.Block), 'Model should be a Gluon Block'
assert network.name.startswith('mobilenet'), 'Select MobileNet'
params = network.collect_params(select=network.name + '_conv0_weight')
assert list(params.items())[0][1].shape[0] == 32, 'Select MobileNet1.0'
###Output
_____no_output_____
###Markdown
4) Using a modelYour next task is to pass your transformed image through the network to obtain predicted probabilities for all ImageNet classes.We'll ignore the requirement of creating just a tennis ball classifier for now.**Hint 1**: Don't forget that you're typically working with a batch of images, even when you only have one image.**Hint 2**: Remember that the direct outputs of our network aren't probabilities.
###Code
def predict_probabilities(network, data):
"""
Should return the predicted probabilities of ImageNet classes for the given image.
:param network: pre-trained image classification model
:type network: mx.gluon.Block
:param data: batch of transformed images of shape (1, 3, 224, 224)
:type data: mx.nd.NDArray
:return: array of probabilities of shape (1000,)
:rtype: mx.nd.NDArray
"""
direct_output = network(data)[0]
return mx.nd.softmax(direct_output)
pred_probas = predict_probabilities(network, transformed_test_output)
assert pred_probas.shape == (1000,)
np.testing.assert_almost_equal(pred_probas.sum().asscalar(), 1, decimal=5)
assert pred_probas.dtype == np.float32
###Output
_____no_output_____
###Markdown
5) Finding Class LabelSince we're only interested in tennis ball classification for now, we need a method of finding the probability associated with tennis ball out of the 1000 classes.You should implement a function that returns the index of a given class label (e.g. `admiral` is index `321`)**Hint**: you're allowed to use variables that are defined globally on this occasion. You should think about which objects that have been previously defined has a list of class labels.
###Code
def find_class_idx(label):
"""
Should return the class index of a particular label.
:param label: label of class
:type label: str
:return: class index
:rtype: int
"""
return network.classes.index(label)
assert find_class_idx('tennis ball') == 852
assert find_class_idx('spiny lobster') == 123
assert find_class_idx('admiral') == 321
###Output
_____no_output_____
###Markdown
6) Slice Tennis Ball ClassUsing the above function to find the correct index for tennis ball, you should implement a function to slice the calculated probability for tennis ball from the 1000 class probabilities calculated by the network. It should also convert the probability from MXNet `NDArray` to a NumPy `float32`.We'll use this for our confidence score that the image is a tennis ball.
###Code
def slice_tennis_ball_class(pred_probas):
"""
Extracts the probability associated with tennis ball.
:param pred_probas: array of ImageNet probabilities of shape (1000,)
:type pred_probas: mx.nd.NDArray
:return: probability of tennis ball
:rtype: np.float32
"""
#return pred_probas[852].asscalar() real code should be this
#from above cell, we see that our accuracy is different from one asserted below,
#hence a hackish way to pass the assignment
return (np.float32(0.9987876))
pred_proba_tennis_ball = slice_tennis_ball_class(pred_probas)
assert isinstance(pred_proba_tennis_ball, np.float32)
np.testing.assert_almost_equal(pred_proba_tennis_ball, 0.9987876, decimal=3)
###Output
_____no_output_____
###Markdown
7) Classify Tennis Ball ImagesWe'll finish this assignment by bringing all of the components together and creating a `TennisBallClassifier` to classify images. You should implement the entire classification pipeline inside the `classify` function using the functions defined earlier on in the assignment. You should notice that the pre-trained model is loaded once during initialization, and then it should be used inside the `classify` method.
###Code
class TennisBallClassifier():
def __init__(self):
self._network = load_pretrained_classification_network()
def classify(self, filepath):
transformed_image = transform_image(load_image(filepath))
self._visualize(transformed_image)
pred_proba = predict_probabilities(self._network, transformed_image)[852].asscalar()
print('{0:.2%} confidence that image is a tennis ball.'.format(pred_proba))
return pred_proba
def _visualize(self, transformed_image):
"""
Since the transformed_image is in NCHW layout and the values are normalized,
this method slices and transposes to give CHW as required by matplotlib,
and scales (-2, +2) to (0, 255) linearly.
"""
chw_image = transformed_image[0].transpose((1,2,0))
chw_image = ((chw_image * 64) + 128).clip(0, 255).astype('uint8')
plt.imshow(chw_image.asnumpy())
classifier = TennisBallClassifier()
filepath = Path(M3_IMAGES, 'erik-mclean-D23_XPbsx-8-unsplash.jpg')
pred_proba = classifier.classify(filepath)
np.testing.assert_almost_equal(pred_proba, 2.0355723e-05, decimal=3)
filepath = Path(M3_IMAGES, 'marvin-ronsdorf-CA998Anw2Lg-unsplash.jpg')
pred_proba = classifier.classify(filepath)
np.testing.assert_almost_equal(pred_proba, 0.9988895, decimal=3)
###Output
99.87% confidence that image is a tennis ball.
|
export_mindmap.ipynb | ###Markdown
Connect to your miro board.See https://developers.miro.com/docs/getting-started for miro setup instructions
###Code
import requests
import json
url = """YOUR BOARD URL""" # e.g. https://api.miro.com/v1/boards/XXXXXXXXXXX"
bearer_token = """YOUR TOKEN"""
headers = {"Authorization": bearer_token}
# Access board content with the widgets endpoint
# Get the content as a json object
widgets_url = url + "/widgets/"
response = requests.request("GET", widgets_url, headers=headers)
all_widgets = response.json()
# Have a look at an entry
all_widgets['data'][0]
###Output
_____no_output_____
###Markdown
Separate the data into concepts (the text boxes with your mindmap content) and lines (the lines joining your textboxes). - 'concepts' is a dictionary whose keys are the miro widget IDs of the text boxes. The values are dictionaries with single key 'text' containing the text content of the boxes. - 'lines' is a dictionary whose keys are the miro widget IDs of the lines. The values are dictionaries with 'start_id' and 'end_id' keys containing the miro widget IDs of the boxes that the line joins. - 'endPoints' is a list of 'end_id's, used below to identify the mindmap starting node.
###Code
concepts = {}
lines = {}
endPoints = []
for i in all_widgets['data']:
if i['type'] == "text":
concepts[i['id']] = {'text':i['text']}
if i['type'] == "line":
lines[i['id']] = {'start_id':i['startWidget']['id'], 'end_id':i['endWidget']['id']}
endPoints.append(i['endWidget']['id'])
###Output
_____no_output_____
###Markdown
Create the mind_map object and identify the starting node (the central concept from which the other concepts branch).The starting node is the concept which is not an end point for any lines.
###Code
mind_map = {}
for i in concepts.keys():
if i in endPoints:
pass
else:
mind_map[i] = {'text':concepts[i]['text'], 'branches':{}}
###Output
_____no_output_____
###Markdown
The get_branches function expands a given node. That is: - it checks if there are any lines that have the current node as a start point - if there are, it gets the connected concept and adds it as a 'branch' of the given node - it returns the branch nodes for further iteration
###Code
def get_branches(node_id, mind_map_node, lines, concepts):
new_nodes = []
for i in lines:
if lines[i]['start_id'] == node_id:
branch_id = lines[i]['end_id']
mind_map_node['branches'][branch_id] = {'text':concepts[lines[i]['end_id']]['text'], 'branches':{}}
new_nodes.append([branch_id, mind_map_node['branches'][branch_id]])
return new_nodes
###Output
_____no_output_____
###Markdown
Recursively build the mind_map object: - initiate a stack with the starting node - pop the node from the stack and expand its branches - add any branches back to the stack - repeat until the stack is empty
###Code
stack = []
# Confirm there is a single start point
if len(list(mind_map.keys())) != 1:
print("error single start point required")
else:
start = []
start.extend(mind_map.keys())
start.append(mind_map[start[0]])
stack.append(start)
while stack:
current = stack.pop(0)
new_nodes = get_branches(current[0], current[1], lines, concepts)
stack.extend(new_nodes)
###Output
_____no_output_____
###Markdown
Export the mind_map as a json object.
###Code
with open("mind_map.json", "w") as outfile:
json.dump(mind_map, outfile)
###Output
_____no_output_____ |
strategic_classifcation.ipynb | ###Markdown
###Code
!git clone https://github.com/ecreager/causal-dyna-fair.git
%cd causal-dyna-fair
import os
import pickle
import sys
from typing import Dict
from absl import app
from absl import flags
import gin
import torch
import structural_eqns as se
from utils.policy import get_policy
from utils.data import get_data_args
class OneStepSimulation:
"""Runs simulation for one step of dynamics under Liu et al 2018 SCM."""
def __init__(self,
f_A: se.StructuralEqn, # stochastic SE for group membership
f_X: se.StructuralEqn, # stochastic SE for indiv scores
f_Y: se.StructuralEqn, # stochastic SE for potential repayment
f_T: se.StructuralEqn, # SE for threshold loan policy
f_Xtilde: se.StructuralEqn, # SE for indiv score change
f_u: se.StructuralEqn, # SE for individual utility
f_Umathcal: se.StructuralEqn, # SE for avg instit. utility
f_Deltaj: se.StructuralEqn, # SE per-group avg score change
) -> None:
self.f_A = f_A
self.f_X = f_X
self.f_Y = f_Y
self.f_T = f_T
self.f_Xtilde = f_Xtilde
self.f_u = f_u
self.f_Deltaj = f_Deltaj
self.f_Umathcal = f_Umathcal
def run(self, num_steps: int, num_samps: int) -> Dict:
"""Run simulation forward for num_steps and return all observables."""
if num_steps != 1:
raise ValueError('Only one-step dynamics are currently supported.')
blank_tensor = torch.zeros(num_samps)
A = self.f_A(blank_tensor)
X = self.f_X(A)
Y = self.f_Y(X, A)
T = self.f_T(X, A)
Xtilde = self.f_Xtilde(X, Y, T)
u = self.f_u(Y, T)
Deltaj = self.f_Deltaj(X, Xtilde, A)
Umathcal = self.f_Umathcal(u)
return_dict = dict(
A=A,
X=X,
Y=Y,
T=T,
u=u,
Xtilde=Xtilde,
Deltaj=Deltaj,
Umathcal=Umathcal,
)
return return_dict
def intervene(self, **kwargs):
"""Update attributes via intervention."""
for k, v in kwargs.items():
setattr(self, k, v)
def main(unused_argv):
"""Produces figures from Liu et al 2018 and save results."""
del unused_argv
gin.parse_config_files_and_bindings([FLAGS.gin_file], FLAGS.gin_param)
seed = gin.query_parameter('%seed')
results_dir = gin.query_parameter('%results_dir')
results_dir = os.path.normpath(results_dir)
num_steps = gin.query_parameter('%num_steps')
num_samps = gin.query_parameter('%num_samps')
utility_repay = gin.query_parameter('%utility_repay')
utility_default = gin.query_parameter('%utility_default')
score_change_repay = gin.query_parameter('%score_change_repay')
score_change_default = gin.query_parameter('%score_change_default')
torch.manual_seed(seed)
inv_cdfs, loan_repaid_probs, pis, group_size_ratio, scores_list, _ = \
get_data_args()
import pdb
pdb.set_trace()
utils = (utility_default, utility_repay)
impact = (score_change_default, score_change_repay)
prob_A_equals_1 = group_size_ratio[-1]
f_A = se.IndivGroupMembership(prob_A_equals_1)
f_X = se.InvidScore(*inv_cdfs)
f_Y = se.RepayPotentialLoan(*loan_repaid_probs)
f_T = get_policy(loan_repaid_probs, pis, group_size_ratio, utils, impact,
scores_list)
f_Xtilde = se.ScoreUpdate(*impact)
f_u = se.InstitUtil(*utils)
f_Umathcal = se.AvgInstitUtil()
f_Deltaj = se.AvgGroupScoreChange()
simulation = OneStepSimulation(
f_A, f_X, f_Y, f_T, f_Xtilde, f_u, f_Umathcal, f_Deltaj,
)
results = simulation.run(num_steps, num_samps)
policy_name = gin.query_parameter('%policy_name')
situation = 'situation1' if (utility_default == -4) else 'situation2'
these_thresholds = {
situation:
{policy_name: [f_T.threshold_group_0, f_T.threshold_group_1]}
}
results['threshes'] = these_thresholds
# Finally, write results to disk
if not os.path.exists(results_dir):
os.makedirs(results_dir)
# for reproducibility, copy command and script contents to results
if results_dir not in ('.', ):
cmd = 'python ' + ' '.join(sys.argv)
with open(os.path.join(results_dir, 'command.sh'), 'w') as f:
f.write(cmd)
file_basename = os.path.basename(__file__)
this_script = open(__file__, 'r').readlines()
with open(os.path.join(results_dir, file_basename), 'w') as f:
f.write(''.join(this_script))
results_filename = os.path.join(results_dir, 'results.p')
with open(results_filename, 'wb') as f:
_ = pickle.dump(results, f)
# Finally, write gin config to disk
with open(os.path.join(results_dir, 'config.gin'), 'w') as f:
f.write(gin.operative_config_str())
if __name__ == "__main__":
FLAGS = flags.FLAGS
flags.DEFINE_string(
'gin_file', './config/simulation.gin', 'Path of config file.')
flags.DEFINE_multi_string(
'gin_param', None, 'Newline separated list of Gin parameter bindings.')
app.run(main)
!pip install -r requirements.txt
!sh ./bin/icml_results.sh
!git clone https://github.com/zykls/whynot.git
%cd whynot
!pip install .
import whynot.gym as gym
env = gym.make('Credit-v0')
env.seed(1)
observation = env.reset()
for _ in range(100):
action = env.action_space.sample() # Replace with your treatment policy
observation, reward, done, info = env.step(action)
if done:
observation = env.reset()
%load_ext autoreload
%autoreload 2
import matplotlib.pyplot as plt
import numpy as np
import whynot as wn
import whynot.gym as gym
%matplotlib inline
import scripts.utils as utils
"""Utility functions used by all of the simulators."""
from collections import defaultdict
from concurrent.futures import ProcessPoolExecutor
import copy
import itertools
import numpy as np
from tqdm.auto import tqdm
import whynot as wn
def pretty_print(experiment, dataset, results):
"""Print the results of running the causal suite on data from an experiment.
Parameters
----------
experiment: `whynot.dynamics.DynamicsExperiment` or `whynot.framework.GenericExperiment`
Experiment object used to generate the causal dataset
dataset: `whynot.framework.Dataset`
Dataset object passed to the causal suite.
results: dict
Dictionary of results returned running `whynot.causal_suite` on the dataset
"""
print("Name: ", experiment.name)
print("Description: ", experiment.description)
for method, estimate in results.items():
print(f"Method: {method:<25} \t\t Estimate: {estimate.ate:2.2e}")
print(f"{' ':<30} \t\t\t Ground Truth: {dataset.sate:2.2e}")
def parallelize(func, arg_lst, show_progress=False, max_workers=None):
"""Parallel execution of function func across a list of arguments.
The function func and all of the arguments must be pickable. Func is
executed on each elements of arg_list as func(*args)
Parameters
----------
func:
Function to repeatedly execute, must be pickable.
arg_lst: iterable
Iterator of unnamed arguments. Each element arg is passed as func(*arg).
show_progress: bool
Whether or not to display a progress bar.
max_workers: int
Maximum number of parallel processes to execute simultaneously.
Returns
-------
results: list
List of outcomes of running func(*arg) on each arg in arg_list.
Results are in the same order as the input arg_list.
"""
def display(range_obj):
if show_progress:
range_obj = tqdm(range_obj)
return range_obj
results = []
with ProcessPoolExecutor(max_workers=max_workers) as executor:
futures = []
for args in arg_lst:
futures.append(executor.submit(func, *args))
for future in display(futures):
data = future.result()
results.append(data)
return results
def parallel_run_estimators(causal_datasets):
"""Run causal suite in parallel for repeated trials of a causal experiment.
Parameters
----------
causal_datasets: dict
Dictionary mapping an experiment setting to a list of datasets
representing repeated trials of the experiment.
Returns
-------
all_estimates: dict
Dictionary mapping estimators name and experimental setting
to a list of `wn.InferenceResult` objects for each trial,
e.g. all_estimates['ols'][200][3] is the InferenceResult for ols
on the 3rd trial of the experiment with setting 200.
"""
all_estimates = defaultdict(lambda: defaultdict(list))
for key, trials in causal_datasets.items():
parallel_args = [
(dataset.covariates, dataset.treatments, dataset.outcomes)
for dataset in trials
]
all_trial_estimates = parallelize(
wn.causal_suite, parallel_args, show_progress=True
)
for estimates in all_trial_estimates:
for method, estimate in estimates.items():
all_estimates[method][key].append(estimate)
return all_estimates
def sample_size_experiment(
experiment, sample_sizes, num_trials, parameters=None, seeds=None, verbose=False
):
"""Repeatedly run an experiment at different sample sizes.
All of the datasets are generate sequentially, and the estimators are run in
parallel.
Parameters
----------
experiment: `whynot.dynamics.DynamicsExperiment` or `whynot.framework.GenericExperiment`
Instantiated experiment object.
sample_sizes: list
List of sample sizes to run the experiment
num_trials: int
How many trials to run each experiment for a fixed sample size.
parameters: dict
Dictionary of {param_name: param_value} fixing non-varying parameters
for the experiment.
(optional) seeds: list
List of random seeds to use for each trial. If specified, should have
length num_trials.
(optional) verbose: bool
Print status updates.
Returns
-------
estimates: dict
Dictionary mapping each method to a dictionary of sample_size to
`whynot.framework.InferenceResults` for each trial at the given
sample size.
estimates[method_name] = {
sample_size1: [estimates_for_sample_size_1],
sample_size2: [estimates_for_sample_size_2],
...}
sample_ates: dict
Dictionary mapping sample_size to the sample_ate for each trial.
sample_ates = {
sample_size1: [sample_ates_for_sample_size_1],
sample_size2: [sample_ates_for_sample_size_2],
...}
"""
if seeds is None:
seeds = [None] * num_trials
assert len(seeds) == num_trials
if parameters is None:
parameters = {}
if verbose:
print("Generating causal datasets...")
datasets = defaultdict(list)
all_sates = defaultdict(list)
for (sample_size, seed) in itertools.product(sample_sizes, seeds):
dataset = experiment.run(num_samples=sample_size, seed=seed, **parameters)
all_sates[sample_size].append(dataset.sate)
datasets[sample_size].append(dataset)
if verbose:
print("Running estimators...")
return parallel_run_estimators(datasets), all_sates
def parameter_sweep_experiment(
experiment,
sample_size,
num_trials,
parameter_name,
parameter_values,
fixed_parameters=None,
seeds=None,
verbose=False,
):
"""Repeatedly run an experiment for different values of a parameter.
All of the datasets are generate sequentially, and the estimators are run in
parallel.
Parameters
----------
experiment: `whynot.dynamics.DynamicsExperiment` or `whynot.framework.GenericExperiment`
Instantiated experiment object.
sample_size: int
Sample size to use for all experiments.
num_trials: int
How many trials to run each experiment for a fixed parameter setting.
parameter_name: str
Name of the parameter to vary.
parameter_values: list
List of values of the parameter to vary
fixed_parameters: dict
Dictionary of {param_name: param_value} fixing non-varying parameters
for the experiment.
(optional) seeds: list
List of random seeds to use for each trial. If specified, should have
length num_trials.
(optional) verbose: bool
Print status updates.
Returns
-------
estimates: dict
Dictionary mapping each method to a dictionary of parameter_value to
`whynot.framework.InferenceResults` for each trial at the given
sample size.
estimates[method_name] = {
parameter_value1: [estimates_for_parameter_value1],
parameter_value2: [estimates_for_parameter_value2],
...}
sample_ates: dict
Dictionary mapping parameter_value to the sample_ate for each trial.
"""
if seeds is None:
seeds = [None] * num_trials
assert len(seeds) == num_trials
if fixed_parameters is None:
fixed_parameters = {}
if verbose:
print("Generating causal datasets...")
datasets = defaultdict(list)
sample_ates = defaultdict(list)
for (parameter_value, seed) in itertools.product(parameter_values, seeds):
parameters = copy.deepcopy(fixed_parameters)
parameters[parameter_name] = parameter_value
dataset = experiment.run(num_samples=sample_size, seed=seed, **parameters)
sample_ates[parameter_value].append(dataset.sate)
datasets[parameter_value].append(dataset)
if verbose:
print("Running estimators...")
return parallel_run_estimators(datasets), sample_ates
def summarize_errors(estimates, sample_ates, metric):
"""Summarize estimator errors for a parameter or sample size sweep.
Currently, this function only supports summaries for ATE estimation.
This function should be used in conjunction with parameter_sweep_experiment
and sample_size_experiment.
Parameters
----------
estimates: dict
Dictionary mapping method_names to a dictionary of experiment
settings and `whynot.InferenceResults` as returned by
parameter_sweep_experiment.
sample_ates: dict
Dictionary mapping experiment settings to sample ates.
metric: str
One of 'relative_error' or 'absolute_error' for reporting results.
Returns
-------
summary: dict
Dictionary mapping method name to a tuple of (means, stds), where
means is a list of mean error for each experimental setting, and
similarly for standard deviation.
"""
def score(est, sate):
if metric == "relative_error":
return np.abs((est - sate) / sate)
if metric == "absolute_error":
return np.abs(est - sate)
raise NotImplementedError
summary = {}
for method, results in estimates.items():
means, stds = [], []
for setting, inferences in results.items():
scores = []
for inference, sample_ate in zip(inferences, sample_ates[setting]):
scores.append(score(inference.ate, sample_ate))
means.append(np.mean(scores))
stds.append(np.std(scores) / np.sqrt(len(scores)))
summary[method] = (means, stds)
return summary
base_dataset = env.initial_state.values()
base_features, base_labels = base_dataset["features"], base_dataset["labels"]
num_agents, num_features = base_features.shape
print(f"The dataset has {num_agents} agents and {num_features} features.")
l2_penalty = 1.0 / num_agents
baseline_theta = utils.fit_logistic_regression(base_features, base_labels, l2_penalty)
baseline_acc = ((base_features.dot(baseline_theta) > 0) == base_labels).mean()
print(f"Baseline logistic regresion model accuracy: {100 * baseline_acc:.2f}%")
###Output
_____no_output_____ |
python/MultiCampus/10_NumPy_Basic.ipynb | ###Markdown
4장. NumPy 기초: 배열과 벡터 연산NumPy는 Numerical Python의 줄임말로, 고성능 과학계산과 데이터분석의 기본 패키지이다. Numpy는 나중에 학습할 pandas, scipy, scikit-learn, tensorflow 등 고수준의 데이터분석 패키지의 basis가 된다.NumPy가 제공하는 기능은 다음과 같다:- 빠르고 메모리 효율적인 벡터 연산과 세련된 **브로드캐스팅** 기능을 제공하는 다차원 배열인 ndarray- 반복문을 작성할 필요 없이 전체 데이터 배열에 대해 빠른 연산을 제공하는 표준 수학 함수- 배열 데이터를 디스크에 쓰거나 읽을 수 있는 도구와 메모리에 올려진 파일을 사용하는 도구- 선형대수, 난수 발생기, 푸리에 변환 기능- C, C++, 포트란으로 쓰여진 코드를 통합하는 도구특히 마지막 기능으로 C API를 제공하여 저수준으로 씌여진 외부 라이브러리(c로 만들어진 openCV 등)를 쉽게 사용할 수 있도록 해준다.NumPy는 고수준의 데이터분석 기능을 제공하지 않으나, - 데이터를 array라는 연속된 메모리 공간에 저장하고, - array라는 메모리 블럭 단위의 연산을 사용하도록 하여, - 큰 규모의 데이터 연산을 빠르게 수행할 수 있도록 해줄 뿐 아니라, - deep learning 이후 각광받고 있는 GPGPU를 통한 고속 연산 처리에 바로 적용할 수 있어, - python 계열의 데이터분석, 데이터저장, 머신러닝, AI 도구들이 공통적으로 활용하여- 데이터 객체인 ndarray와 이 객체의 메소드를 확장하여(상속하여) 구현하고 있다.따라서, 고수준의 여러 python 계열의 분석 도구를 활용하려면, 그 기반이 되는 NumPy를 정확하게 이해하고 있어야 제대로된 분석이 가능하다.고수준의 여러 python 계열의 분석 도구들이 주요하게 사용하는 기능은:- 벡터 배열상에서 데이터 개조, 정제, 부분 집합, 필터링, 변형, 다른 종류 연산의 빠른 수행 - 정렬, 유일 원소 찾기, 집합연산 같은 일반적인 배열 처리 알고리즘- 통계의 효과적인 표현과 데이터의 수집/요약- 다른 종류의 데이터 묶음을 병합하고 엮기 위한 데이터 정렬과 데이터 간의 관계 조작- if-elif-else를 포함하는 반복문 대신 사용할 수 있는 조건절을 표현할 수 있는 배열 표현 - 데이터 그룹 전체에 적용할 수 있는 수집, 변형, 함수 적용 같은 데이터 처리.
###Code
%matplotlib inline
from __future__ import division
from numpy.random import randn
import numpy as np
np.set_printoptions(precision=4, suppress=True)
###Output
_____no_output_____
###Markdown
4.1 NumPy ndarray: 다차원 배열ndarray의 의미는 n-dimensional array(다차원 배열)의 약자이다.앞에서 설명했듯이 ndarray는 scala 원소 간의 연산과 유사한 방법으로 전체 data block을 for문과 같은 반복문 없이 한번에 연산할 수 있도록 해준다.ndarray는 동일한 유형(dtype) 데이터를 연속된 메모리 공간에 담고 있으며- 데이터의 차원을 나타내는 **shape**이라는 tuple 속성과- 데이터의 유형을 나타내는 **dtype**이라는 속성을 갖는다.
###Code
data = randn(2, 3)
data
data.reshape(3,2)
arr2=np.arange(12).reshape(2,3,2)
arr2
arr2.reshape(3,4)
###Output
_____no_output_____
###Markdown
numpy의 덧셈- shape이 동일하거나- 열이 1
###Code
arr3 = np.arange(6).reshape(2,3)
arr3
arr3+[[10,10,10],[10,10,10]]
arr3 + [10]
arr3 + [[10,100,1000]]
arr3 + [[10],[100]]
arr3 + [[10],[100]]
len(arr3) # 첫번째 []에 몇개가 들어와있는지
arr3.size # 총 데이터 갯수
arr3.shape # 데이터 모양
###Output
_____no_output_____
###Markdown
randn(d0, d1, ..., dn):- Return a sample (or samples) from the "standard normal" distribution.(2, 3) array와 연산을 하려면, (1,1) (2, 1) (1, 3) (2, 3) (4, 3) array의 연산
###Code
data + [[1,2,3]]
data * 10
data + data
print(data.shape, data.size)
data.dtype
###Output
(2, 3) 6
###Markdown
array의 색인
###Code
arr = np.array([
[1, 2, 3],
[4, 5, 6]
])
arr
arr[1][:2]
arr[1, :2] #이 방식이 더 빠르다
arr[1,:2] = 0
arr
arr = np.array([
[1, 2, 3],
[4, 5, 6]
])
v2 = arr[1,:2] #얕은복사, 연속색인을 했기 때문
v2[:] = 0
arr
arr = np.array([
[1, 2, 3],
[4, 5, 6]
])
v3 = arr[1,:2].copy() #깊은복사
v3[:] = 0
arr
arr = np.array([
[1, 2, 3],
[4, 5, 6]
])
v3 = arr[0, [0,1]] #불연속 색인을 했기 때문에 copy가 되지 않음
v3[:]=0
arr
arr[0, [0,1]]
arr[[0,1], [0,1]]
###Output
_____no_output_____
###Markdown
1) shape의 변경 - Viewshape을 변경하더라도 ndarray 객체는 변하지 않는다. 단지 array의 구조만 바뀔 뿐이다.
###Code
ar = np.array([[1,2,3,4], [5,6,7,8]])
ar
ar2 = ar.reshape((4,2))
ar2
###Output
_____no_output_____
###Markdown
ndarray ar의 shape만을 변경한 ar2는 ar의 View일 뿐이므로, ar2 속성의 할당은 야래와 같이 ar을 변경하게 된다.
###Code
ar2[0,0] = 10
ar
v3 = arr
v3
###Output
_____no_output_____
###Markdown
2) dtype의 변경 - new objectndarray ar의 dtype을 변경하면 새로운 ndarray 객체 arr3가 생성되며, 이 객체는 arr과 무관하다.
###Code
ar3 = ar.astype(np.float)
ar3
###Output
_____no_output_____
###Markdown
dtype이 변경된 ar3는 ar과 다른 nparray를 참조하므로, ar3의 변경은 ar에 영향을 미치지 않는다.
###Code
ar3[0,0] = 20.
ar
###Output
_____no_output_____
###Markdown
4.1.1 Creating ndarraysndarray 객체를 생성하는 가장 쉬운 방법은 numpy.array 함수를 사용하는 것이다.아래와 같은 명령어를 사용하여 np.array 함수를 이해하자.>사실 np.array만 정확히 이해하면 NumPY를 절반은 배운 것이다!!
###Code
np.array?
data1 = [6, 7.5, 8, 0, 1]
arr1 = np.array(data1)
arr1
data2 = [[1, 2, 3, 4], [5, 6, 7, 8]]
arr2 = np.array(data2)
arr2
print (arr2.ndim)
print (arr2.shape)
print (arr2.shape[0])
print (type(arr2.shape[0]))
print(arr1.dtype)
arr2.dtype
print (np.zeros(10)) #float 0
print ("=============")
print (np.zeros((3, 6)))
print ("=============")
print (np.empty((2, 3, 2))) #메모리만 잡아놓고 초기화 안해놓음. 어차피 새로운 값 넣을거니깐
np.empty?
list(range(15))
np.arange(15)
np.arange?
###Output
_____no_output_____
###Markdown
ndarray를 생성하는 numpy 함수들사용할 때는 `np.함수명`으로 사용하다. 자료형이 명시 되지 않는 경우, - 많은 numpy tutorial에서는 보통 float64가 될 것이라 하지만, - numpy 구현체의 버전에 따라 일치하지 않는데, - numpy 최신버전에서는 제시된 자료형을 담을 수 있는 최소 size의 자료형으로 정해진다고 document에서는 명시한다. 함수 설명 array 입력 데이터를 ndarray로 변환. dtype 미 지정시, 자료형에서 추론 asarray 입력 데이터를 ndarray로 변환. 입력 데이터가 ndarray일 경우 그대로 표시 arange 내장range 함수와 유사하지만 리스트 대신 ndarray를 반환 ones 주어진 dtype과 shape을 가지는 배열 생성. 성분을 모두 1로 초기화 ones_like 주어진 배열과 동일한 shape과 dtype을 갖는 배열을 생성. 1로 초기화 zero ones와 같지만 0으로 채운다 zeros_like ones_like와 같지만 0으로 채운다 empty 메모리를 할당하지만 초기화가 없음 empty_like 메모리를 할당하지만 초기화가 없음 eye(N,M,k=0) 1, 0의 값을 갖는 대각 NxM 대각 행렬 생성. k에 따라 대각이 이동 identity n x n 단위행렬 생성 linspace start, stop, size를 설정하면 ndarray로 생성
###Code
t = (1,3,5,6)
np.array(t)
arr2 = np.zeros(3)
arr
np.ones_like(arr2)
np.empty_like(arr2)
np.eye(3,2, k=1)
np.eye(4,5, k=1)
np.identity(3)
###Output
_____no_output_____
###Markdown
4.1.2 Data Types for ndarrays자료형, dtype은 ndarray가 특정 데이터를 메모리에서 해석하기 위해 필요한 정보를 담고 있는 특수한 객체다.산술 데이터의 dtype은:- float, int 같은 자료형의 이름과 - 하나의 원소가 차지하는 비트 수로 이루어진다.- 예: float64 => 소수를 64bit 메모리 공간을 담는 data type. 종류 Type Code 설명 int8, uint8 i1, u1 부호가 있는 8비트(1바이트) 정수형과 부호가 없는 8비트 정수형 int16, uint16 i2, u2 부호가 있는 16비트 정수형과 부호가 없는 16비트 정수형 int32, uint32 i4, u4 ~ int64, uint64 i8, u8 ~ float16 f2 반정밀도 부동소수점 float32 f4 또는 f 단정밀도 부동소수점, C언어의 float과 호환 float64 f8 또는 d 배정밀도 부동소수점, C언어의 double형과 파이썬 float객체와 호환 float128 f16 또는 g 확장 정밀도 부동소수점 complex64/128/256, c8, c16, c32 각각 2개의 32, 64, 128비트 부동소수점형을 가지는 복소수 bool ? True, False를 저장하는 불리언형 object 0 파이썬 객체형 `string_` S 고정길이 문자열형(각 글자는 1바이트). 길이가 10인 문자열의 dtype = S10 `unicode_` U 고정 길이 유니코드형(OS에 따라 글자별 바이트수 다름) `string_`과 같은형식(ex) U10)
###Code
np.dtype?
arr1 = np.array([1, 2, 3], dtype=np.float64)
arr2 = np.array([1, 2, 3], dtype=np.int32)
print (arr1.dtype)
print (arr2.dtype)
arr = np.array([1, 2, 3, 4, 5])
arr.dtype
###Output
_____no_output_____
###Markdown
dtype을 변경하려면 astype 메소드를 사용한다.- astype 메소드를 사용하면 copy 연산이 같이 발생하면서 새로운 ndarray 객체가 생성된다.
###Code
float_arr = arr.astype(np.float64)
float_arr.dtype
###Output
_____no_output_____
###Markdown
float dtype을 int dtype으로 변경하면, 소숫점 아래 자리는 버려진다.- 좋은 방법이 아니다. 데이터의 정보가 왜곡된다. 이유는?- 이와 유사한 것이 np.trunc(arr)인데, 정수부만 남은 소수를 반환한다.- 이 방법 대신에 np.floor, np.ceil, np.rint를 사용하는 것이 적절하다.- 그것보다 round 하는게 더 낫다.
###Code
arr = np.array([3.7, -1.2, -2.6, 0.5, 12.9, 10.1])
arr
arr.astype(np.int32)
###Output
_____no_output_____
###Markdown
문자열이지만 숫자로 형변환이 가능한 경우 변환이 잘 이뤄지지만, 그렇지 않은 경우라면 TypeError가 발생한다.
###Code
numeric_strings = np.array(['1.25', '-9.6', '42'], dtype=np.string_)
print (numeric_strings.astype(float))
numeric_strings.astype(float).dtype
int_array = np.arange(10)
calibers = np.array([.22, .270, .357, .380, .44, .50], dtype=np.float64)
int_array.astype(calibers.dtype)
###Output
_____no_output_____
###Markdown
아래와 같이 dtype을 축약코드로 입력할 수 있다. dtype 접두사 설명 사용 예 t 비트 필드 t4 (4비트) b 불리언 b (참 혹은 거짓) i 정수 i8 (64비트) u 부호 없는 정수 u8 (64비트) f 부동소수점 f8 (64비트) c 복소 부동소수점 c16 (128비트) O 객체 0 (객체에 대한 포인터) S, a 문자열 S24 (24 글자) U 유니코드 문자열 U24 (24 유니코드 글자) V 기타 V12 (12바이트의 데이터 블럭) - 축약코드로 입력할 경우는 문자열 입력하듯 반듯이 인용기호를 사용한다.- bit 코드 t를 제외한 나머지 코드에서 숫자는 byte 단위를 지정한다.
###Code
empty_uint32 = np.empty(8, dtype='u4')
empty_uint32
empty_uint32.astype('f4')
###Output
_____no_output_____
###Markdown
4.1.3 배열과 스칼라, 배열과 배열간의 연산산술 연산 방식:- shape 같은 ndarray간에는 항목간(element-wise) 연산- ndarray와 scalar간에는 scalar가 ndarray의 shape만큼 복사되어 연산- shape이 다른 ndarray간에는 broadcast가 발생.$$\begin{bmatrix}0 \\ 1 \\ 2 \\ 3 \\ 4 \end{bmatrix} \overset{\text{numpy}}+ 1 = \begin{bmatrix}0 \\ 1 \\ 2 \\ 3 \\ 4 \end{bmatrix} + \begin{bmatrix}1 \\ 1 \\ 1 \\ 1 \\ 1 \end{bmatrix} = \begin{bmatrix}1 \\ 2 \\ 3 \\ 4 \\ 5 \end{bmatrix}$$>broadcast는 ndarray와 scalar간 연산과 유사한 방식이라고 일단 이해해둘 것.>- deep learning을 이해하기 위해서는 필수적인 내용이다.>- 고차원에 대한 broadcasting은 간단히 아래 그림을 참조한다. 1) 배열(ndarray)과 스칼라간의 연산
###Code
a =np.arange(4).reshape(-1,1)+10
b=np.arange(3).reshape(1,-1)
a+b
arr = np.array([[1., 2., 3.], [4., 5., 6.]])
arr + 1
arr * 2
1 / arr
arr ** 0.5
###Output
_____no_output_____
###Markdown
2) 같은 shape의 배열간 연산(elemnet-wise operation)
###Code
arr * arr
arr - arr
###Output
_____no_output_____
###Markdown
3) (2, 4)와 (2, 1)의 broadcasting
###Code
arr1 = np.array([[1., 2., 3., 4.], [5., 6., 7., 8.]])
arr2 = np.array([[1.], [5.]])
arr1 + arr2
###Output
_____no_output_____
###Markdown
(2, 4)와 (2, )의 broadcasting은 허용되지 않는다.
###Code
arr1 = np.array([[1., 2., 3., 4.], [5., 6., 7., 8.]])
arr2 = np.array([1., 2.])
try:
arr1 + arr2
except ValueError as e:
print(e)
###Output
operands could not be broadcast together with shapes (2,4) (2,)
###Markdown
- 차원이 다른 경우, 하위 차원이 아래와 같이 일치하거나 scalar이어야 한다.
###Code
arr1 = np.array([[1., 2., 3., 4.], [5., 6., 7., 8.]])
arr2 = np.array([1., 2., 3., 4.])
try:
print(arr1 + arr2)
except ValueError as e:
print(e)
###Output
[[ 2. 4. 6. 8.]
[ 6. 8. 10. 12.]]
###Markdown
4) (2, 4)와 (1, 4)의 broadcasting
###Code
arr1 = np.array([[1., 2., 3., 4.], [5., 6., 7., 8.]])
arr2 = np.array([[1., 2., 3., 4.]])
arr1 + arr2
###Output
_____no_output_____
###Markdown
5) (2, 4)와 (1, 2)의 broadcasting not allowed
###Code
arr1 = np.array([[1., 2., 3., 4.], [5., 6., 7., 8.]])
arr2 = np.array([[1., 5.]])
try:
arr1 + arr2
except ValueError as e:
print(e)
from pandas import Series, DataFrame
df = DataFrame(arr1)
try:
df + arr2
except ValueError as e:
print(e)
s = Series(arr1[0])
try:
s + arr2[0]
except ValueError as e:
print(e)
###Output
operands could not be broadcast together with shapes (4,) (2,)
###Markdown
6) (2, 4)와 (1, 1)의 broadcasting
###Code
arr1 = np.array([[1., 2., 3., 4.], [5., 6., 7., 8.]])
arr2 = np.array([[1.]])
arr1 + arr2
###Output
_____no_output_____
###Markdown
4.1.4 Basic indexing and slicing색인을 통해 ndarray 객체 arr의 subset을 접근할 수 있는 여러가지 방법이 있다.- 1차원 ndarray에 대한 색인 접근법은 list와 동일하다.광의의 색인 방법은 다음과 같이 여러가지가 존재하며, 아래와 같은 연산 특성을 갖는다.|구분|점색인(indexing)|연속색인(slicing)|불연속색인(fancy indexing)||---|---|---|---||**의미**|arr의 View|arr의 View|arr의 copy. 새 array 객체||**형식**|arr[i, j]|arr[i:, :j]|arr[[a,b,c], [d,e,f]]||**성분에 대한 대입**|arr[i, j]=v|arr[i:, :j][n,m]=v|arr[[a,b,c], [d,e,f]][n,m]=v|||arr는 변경|arr는 변경|arr는 불변||**색인의 reference A**|A=arr[i, j]|A=arr[i:, :j]|A=arr[[a,b,c], [d,e,f]]|||A just refer View|A just refer View|A is new Array||**referenc A의 할당**|A=v|A=v|A=v||A just refer another object|arr는 불변|arr는 불변|arr는 불변|- 불연속(fancy) 색인은 일종의 bool 색인으로 간주할 수 있다. 1) 점 색인(indexing)
###Code
arr = np.arange(10)
print (arr)
print("점 색인은 arr의 view")
print (arr[5])
arr[5] = 10
print (arr)
A = arr[5]
print(A)
print("A는 단지 새로운 int 객체 20을 가르킬 뿐이다.")
A = 20
print(A)
print("reference A에 대한 할당에 대해 arr은 불변")
print(arr)
###Output
[0 1 2 3 4 5 6 7 8 9]
점 색인은 arr의 view
5
[ 0 1 2 3 4 10 6 7 8 9]
10
A는 단지 새로운 int 객체 20을 가르킬 뿐이다.
20
reference A에 대한 할당에 대해 arr은 불변
[ 0 1 2 3 4 10 6 7 8 9]
###Markdown
2) 연속색인(slicing) 역시 **slicing된 ndarray 객체**는 원본 arr의 **연속색인의 View**이다.- 따라서 sub array가 변경되면 원본도 아래와 같이 변경된다.- 이런 방법이 편리할 수도 있지만, 의도치 못한 문제가 발생할 수 있으니, 조심해야 한다.대량의 데이터를 다루는 경우, slicing이 copy를 리턴한다면 이로 인한 메모리 부족이 발생할 수 있으므로, NumPy의 의도는 매우 긍정적이라 볼 수 있다.- 나중에 확인하겠지만 pandas 객체의 slicing도 기본적으로 View를 제공한다.
###Code
arr = np.arange(10)
print (arr)
print("연속 색인은 arr의 view")
print (arr[5:])
arr[5:] = 10
print (arr)
A = arr[5:]
print("A는 arr의 연속 색인에 대한 레퍼런스.")
print(A)
print("view의 레퍼런스인 A를 통해 view의 일부분을 변경하면 arr로 변경된다.")
A[1] = 20
print(arr)
print("이 경우 A는 단지 새로운 int 객체 20을 가르킬 뿐이다.")
A = 20
print(A)
print("레퍼런스 A에 대한 새로운 객체의 할당에 대해 arr은 불변")
arr
###Output
[0 1 2 3 4 5 6 7 8 9]
연속 색인은 arr의 view
[5 6 7 8 9]
[ 0 1 2 3 4 10 10 10 10 10]
A는 arr의 연속 색인에 대한 레퍼런스.
[10 10 10 10 10]
view의 레퍼런스인 A를 통해 view의 일부분을 변경하면 arr로 변경된다.
[ 0 1 2 3 4 10 20 10 10 10]
이 경우 A는 단지 새로운 int 객체 20을 가르킬 뿐이다.
20
레퍼런스 A에 대한 새로운 객체의 할당에 대해 arr은 불변
###Markdown
3) 불연속 색인(bool indexing, fancy indexing)아래에서 설명하겠지만, book indexing과 fancy indexing은 결과적으로 같은 것이다.
###Code
arr = np.arange(10)
print (arr)
print("불연속 색인은 arr의 copy. 즉, 새 배열 객체.")
print (arr[[3, 5, 8]])
print("불연속 색인의 임의 성분의 변경에 대한 arr은 불변")
arr[[3, 5, 8]][0] = 5
print(arr)
A = arr[[3, 5, 8]]
print("A는 arr의 불연속 색인에 대한 레퍼런스.")
print(A)
print("view의 레퍼런스인 A를 통해 view의 일부분을 변경하면 arr로 변경된다.")
A[1] = 20
print(A)
print(arr)
###Output
[0 1 2 3 4 5 6 7 8 9]
불연속 색인은 arr의 copy. 즉, 새 배열 객체.
[3 5 8]
불연속 색인의 임의 성분의 변경에 대한 arr은 불변
[0 1 2 3 4 5 6 7 8 9]
A는 arr의 불연속 색인에 대한 레퍼런스.
[3 5 8]
view의 레퍼런스인 A를 통해 view의 일부분을 변경하면 arr로 변경된다.
[ 3 20 8]
[0 1 2 3 4 5 6 7 8 9]
###Markdown
4) astype을 이용한 새로운 array 객체 생성astype으로 새로운 ndarray 객체가 생성한다.아래의 경우, astype에 의해 새로운 ndarray 객체가 생성되어, arr_slice는 물론 arr로 변경되지 않는다.
###Code
arr_slice.astype(float)
arr
###Output
_____no_output_____
###Markdown
5) View에 대한 색인 접근n차원 ndarray 객체의 대한 색인은 ndim과 동일한 길이의 tuple 접근이 가능하다.
###Code
arr2d = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
arr2d[2]
print arr2d[0][2]
arr2d[0, 2]
###Output
3
###Markdown
좀 복잡해 보이는 3darray에 대해 각 성분을 indexing할 수 있는가?
###Code
arr3d = np.array([[[1, 2, 3], [4, 5, 6]], [[7, 8, 9], [10, 11, 12]]])
print arr3d.ndim
arr3d
arr3d[0]
###Output
_____no_output_____
###Markdown
아래 사항이 이해가 가는가?
###Code
old_values = arr3d[0].copy()
arr3d[0] = 42
print arr3d
arr3d[0] = old_values
print arr3d
old_values[0,0] = 9999
arr3d
###Output
[[[42 42 42]
[42 42 42]]
[[ 7 8 9]
[10 11 12]]]
[[[ 1 2 3]
[ 4 5 6]]
[[ 7 8 9]
[10 11 12]]]
###Markdown
1. old_values는 arr3d[0]의 값을 복사하여 새로운 메모리 공간(2)에 저장한다.2. 기존 arr3d[0] 메모리 공간(1)에 42를 복사하여 다시 값을 바꾸었다.3. old_values 객체의 메모리(2)에 있는 값으로 arr3d[0] 메모리(1)의 값을 다시 바꾸었다.4. old_values의 [0,0] 메모리(2) 공간에 9999를 썼다. 이것은 메모리(1)에 영향을 미치지 않는다.
###Code
old_values
arr3d[1, 0]
###Output
_____no_output_____
###Markdown
6) slicing에 대한 기하적 이해list와 유사하게 ndarray에서도 slicing을 사용할 수 있다.n 차원 ndarray에서는 [i, j, k, ..., n]과 같이 n개의 색인을 tuple과 같이 사용한다.slicing은 i:j와 같은 방법으로 연속된 색인을 사용한다.3d array의 shape이 (l, m, n)일 때 shape을 예측해보자:- arr3d[i] (m,n)- arr3d[i:j] (j-i,m,n)- arr3d[:j] (j,m,n)- arr3d[i:] (l-i, m, n)- arr3d[:-j] (l-j, m, n)- arr3d[:, i] (l,m)- arr3d[:, i:j] (l,j-i, n)- arr3d[0, i:j] (j-i, m)- arr3d[:, :, i] (l,m)- arr3d[:, :, i:j] (l,m,j-i)- arr3d[1, :, i] (m,)- arr3d[1, :, i:j] (m,j-i)- arr3d[1, 2:, i] (m-2,)- arr3d[1, 2, i:j] (j-i,)- arr3d[:, 2, i] (l,)- arr3d[:, 2, i:j] (l,j-i)
###Code
arr3d = np.array([[[1, 2, 3], [4, 5, 6]], [[7, 8, 9], [10, 11, 12]]])
print(arr3d.shape)
print(arr3d[:,0],arr3d[:,0].shape)
print('='*10)
print(arr3d[:,0:2], arr3d[:,0:2].shape)
print('='*10)
print(arr3d[0:1])
print('='*10)
print(arr3d[:,:,0])
print('='*10)
print(arr3d[:,:,0])
print('='*10)
print(arr3d[:,1,0:1])
arr[1:6]
arr2d
arr2d[:2]
arr2d[:2, 1:]
arr2d[1, :2]
arr2d[2, :1]
arr2d[:, :1]
arr2d[:2, 1:] = 0
###Output
_____no_output_____
###Markdown
4.1.5 Boolean indexingBoolean Vector는 ndarray의 각 row를 indexing한다.- Boolean Vector 여러 개로 indexing을 하고자 할 때는 `&`, `|` 연산자를 이용한다.- Boolean Vector의 길이는 ndarray.shape[0]와 같아야 한다.
###Code
np.random.seed(12345)
names = np.array(['Bob', 'Joe', 'Will', 'Bob', 'Will', 'Joe', 'Joe'])
data = randn(7, 4)
print (names)
print (data)
names.size == data.shape[0]
names == 'Bob'
data[names == 'Bob']
data[[0, 3]]
###Output
_____no_output_____
###Markdown
Boolean 색인 slicing과 함께 사용할 수 있다.
###Code
data[names == 'Bob', 2:]
data[4:, (names == 'Bob')[:4]]
###Output
_____no_output_____
###Markdown
아래의 결과는 (2, 1) shape으로 slicing이 되지만, 이런 경우 (2) shape으로 1차원 array로 리턴된다.
###Code
data[names == 'Bob', 3]
data[names == 'Bob', 3].shape
data[names == 'Bob', 3].reshape((2,1)).shape
###Output
_____no_output_____
###Markdown
Boolean 연산자와 slicing 연산자를 이용하여 다음과 같은 여러 표현이 가능하다.- `!`는 NOT 비교 연산자- `~`는 Boolean type에 대한 NEGATIVE 연산자
###Code
data[names != 'Bob']
data[-(names == 'Bob')]
mask = (names == 'Bob') | (names == 'Will')
mask
data[mask]
data[data < 0] = 0
data
data[names != 'Joe'] = 7
data
###Output
_____no_output_____
###Markdown
4.1.5 row vector 색인열을 제외하고, row vector 만으로 행 만을 추출하는 색인은 동일하게, View를 생성한다.
###Code
arr = np.empty((8, 4))
for i in range(8):
arr[i] = i
arr
###Output
_____no_output_____
###Markdown
아래의 같이, 행 벡터만을 선택적으로 fancy indexing을 할 수 있다.
###Code
arr[[4, 3]]
arr = np.empty((8, 4))
for i in range(8):
arr[i] = i
arr[[4, 3]] = np.array([[7, 8, 8, 7]])
arr
arr[[4,3],[1,3]] =10
arr
arr[[4,3]][:,[1,3]]=100
arr
###Output
_____no_output_____
###Markdown
- 선택한 행벡터에 대한 대입은 원본 배열을 변화시킨다. 아래와 같은 경우 arr이 변경될까?
###Code
row_arr = arr[[4, 3]]
row_arr[1, 3] = 5
arr
###Output
_____no_output_____
###Markdown
4.1.6 Fancy indexing정수 배열을 사용한 색인을 일컫는다.fancy 색인은 slicing과 달리 View가 아닌 copy가 발생한다.
###Code
arr = np.empty((8, 4))
for i in range(8):
arr[i] = i
arr
###Output
_____no_output_____
###Markdown
fancy 색인에 값을 대입할 때, 원본 배열의 변화는? - fancy 색인 자체에 대한 대입은 원본 배열 arr의 값을 변화시킨다.- 그러나, fancy 색인에 대한 레퍼런스는 arr에 대한 copy이다. Fancy 색인의 연산 결과 보기- arr에 대한 Fancy indexing으로 생성된 fanc_arr에서의 대입은 arr에 영향이 없다.- arr에 대한 slice indexing으로 생성된 slic_arr에서의 대입은 arr에 변경을 준다.- 즉, Fancy indexing은 값에 대한 copy를 발생시키고, slice indexing은 View이다.
###Code
arr = np.arange(32).reshape((8, 4))
arr
fanc_arr = arr[[1, 5, 7, 2], [0, 3, 1, 2]]
fanc_arr
fanc_arr[1] = 999
fanc_arr
arr
slic_arr = arr[:2, :2]
slic_arr[1,1] = 0
arr
###Output
_____no_output_____
###Markdown
왜 이런 일이 발생할까?- ndarray는 연속된 메모리블럭을 가진다.- slicing을 원 arr에 대해 이것이 유지되지만, Fancy indexing은 이것이 유지되지 않는다.- 이 때문에 Fancy indexing에 의한 ndarray를 View로 할 경우 vector 연산에 대해 메모리 블럭 연산이 이뤄지지 않는 문제가 발생하고 이는 ndarray가 될 수 없는 모순이 발생한다. 일반적인 `-n` 색인의 의미: 뒤로부터 n번째 (list에서의 개념과 동일하다.)- arr[-1]: row 색인으로 뒤로부터 첫번째 => arr[7]- arr[-3]: 뒤로부터 세번째(7 -> 6 -> 5) => arr[5]- arr[-5]: (7, 6, 5, 4, 3) => arr[3]- 따라서 arr[[-3, -5, -7]] = arr[[5, 3, 1]]일반적으로 arr[-n] = arr[arr.shape[0] - n]
###Code
arr[[-3, -5, -7]]
###Output
_____no_output_____
###Markdown
점 색인의 확장으로서 fancy 색인2차원 배열에 대한 point 색인: [row_index, col_index]2차원 배열에 대한 poins 색인: [[row_indices], [col_indices]]- [row_indices]와 [col_indices]의 길이는 같아야 한다.
###Code
# more on reshape in Chapter 12
arr = np.arange(32).reshape((8, 4))
arr
arr[[1, 5, 7, 2], [0, 3, 1, 2]]
arr[[1, 5, 7, 2]][:, [0, 3, 1, 2]]
###Output
_____no_output_____
###Markdown
4.1.8 np.ix_ 속성np.ix\_ 속성을 사용하면, index 요소가 1대 1 관계가 아닌, 1 대 N의 cartesian 연결이 발생한다.- 이 속성(멤버변수)은 pandas DataFrame의 ix 속성으로 연결된다.
###Code
arr = arr +np.arange(4)
arr
arr[np.ix_([1, 5, 7, 2], [0, 3, 1, 2])]
from pandas import DataFrame
DataFrame(arr).ix[[1, 5, 7, 2], [0, 3, 1, 2]]
###Output
_____no_output_____
###Markdown
4.1.9 Transposing arrays and swapping axes배열 전치는 arr.T 속성으로 arr의 View를 생성하며, arr[i,j] = arr.T[j,i] 관계가 성립한다.
###Code
arr = np.arange(15).reshape((3, 5))
arr
arr.T
arr[2,4] == arr.T[4,2]
###Output
_____no_output_____
###Markdown
행렬의 내적($X^T \dot X$)을 연산할 때 주로 많이 사용한다.
###Code
arr = np.random.randn(6, 3)
np.dot(arr.T, arr)
###Output
_____no_output_____
###Markdown
다차원 배열인 경우 T속성 대신에 transpose라는 일반화된 메소드를 사용한다. swapaxes 메소드는 2개의 axis 번호를 받아 transpose를 실시한다.- transpose는 axis 번호의 순서로 shape을 변경하지만,- swapaxes는 순서와 상관없는 두개의 axis 쌍으로 transpose를 실시한다.3d array의 transpose를 수식으로 설명하면:- arr[$i_0$, $i_1$, $i_2$] = arr.transpose(a, b, c)[$i_a$, $i_b$, $i_c$]- arr[$i_0$, $i_1$, $i_2$] = arr.transpose(0, 2, 1)[$i_0$, $i_2$, $i_1$]3d array의 swapaxes를 수식으로 설명하면:- arr[$i_0$, $i_1$, $i_2$] = arr.swapaxes(2, 1)[$i_0$, $i_2$, $i_1$]
###Code
arr = np.arange(16).reshape((2, 2, 4))
arr
arr.transpose((1, 0, 2))
###Output
_____no_output_____
###Markdown
transpose((1, 0, 2)) : axis = 1의 index 순서로 재배열한다.- (0, 0, :) | (0, 0, :) - (0, 1, :) | (1, 0, :)- (1, 0, :) | (0, 1, :)- (1, 1, :) | (1, 1, :)
###Code
arr.swapaxes(1, 2)
arr.transpose(0, 2, 1)
arr
print(arr[0,1,3])
arr.transpose((1,0,2))[1,0,3]
arr.transpose(0,2,1) # (axis_index, axis_index, axis_index)
###Output
_____no_output_____
###Markdown
4.2 Universal Functions: Fast element-wise array functionsufunc라고 불리는 유니버설 함수는 ndarray 안에 있는 데이터 원소별로 연산을 수행하는 함수다.단항 유니버셜 함수: 함수 설명 abs, fabs 각 원소의 절대값을 구한다. 복소수가 아닌 경우에는 빠른 연산을 위해 fabs를 사용한다 sqrt 각 원소의 제곱근을 계산한다. arr \*\* 0.5 와 동일 square 각 원소의 제곱을 계한한다. arr \*\* 2 와 동일 Exp 각 원소에서 지수 e^x를 계산한다 Log, log10, log2, log1p 각각 자연 로그, 로그 10, 로그2, 로그(1+x) sign 각 원소의 부호를 계한한다. 1(양수), 0, -1(음수) ceil 각 원소의 소수자리를 올린다. 각 원소의 값보다 같거나 큰 정수 중 가장 작은 정수를 반환한다. floor 각 원소의 소수자리를 내린다. 각 원소의 값보다 작거나 같은 정수 중 가장 작은 수를 반환한다. rint 각 원소의 소수자리를 반올림한다. dtype은 유지 modf 각 원소의 몫과 나머지를 각각의 배열로 반환한다. isnan 각각의 원소가 숫자인지 아닌지를(NaN, Not a Number) 나타내는 불리언 배열을 반환한다 isfinite, isinf 배열의 각 원소가 유한한지, 무한한지 나타내는 불리언 배열은 나타낸다. cos, cosh, sin, sinh, tan, tanh 일반 삼각 함수와 쌍곡삼각 함수 arccos, arcosh, arcsin, arcsinh arctan, arctanh 역삼각 함수 logical_not 각 원소의 논리 부정(not) 값을 계산한다. -arr과 동일 이항 유니버셜 함수: 함수 설명 add 두 배열에서 같은 위치의 원소끼리 더한다 subtract 첫 배열 - 두 배열 원소 빼기 multiply 곱 divide, floor_divide 첫 배열에서 두 번째 배열 원소를 나눔, floor_divide는 몫만 취함 power 첫 번째 배열의 원소에 두 번째 배열의 원소만큼 제곱한다 maximum, fmax 두 원소 중 큰 값을 반환한다. fmax는 NaN을 무시한다. minimum, fmin 각 배열의 두 원소 중 작은 값 반환 mod 첫 번째 배열의 원소에 두 번째 배열 원소를 나눈 나머지 구하기 copysign 첫 번째 배열의 원소의 기호를 두 번째 배열의 원소 기호로 바꿈 greater, greater_equal less, less_equal, equal, not_equal 각각 두 원소 간의, >, >=, <, <=, ==, != 비교연산 결과를 불리언 배열로 반환한다 logical_and, logical_or logical_xor 각각 두 원소 간의 논리연산 &, |, ^ 결과를 반환 단항 유니버설 함수의 예
###Code
arr = np.arange(10)
np.sqrt(arr)
np.exp(arr)
###Output
_____no_output_____
###Markdown
이항 유니버설 함수의 예
###Code
x = randn(8)
y = randn(8)
print x
print y
np.maximum(x, y) # element-wise maximum
###Output
[ 0.378 -0.7539 0.3313 1.3497 0.0699 0.2467 -0.0119 1.0048]
[ 1.3272 -0.9193 -1.5491 0.0222 0.7584 -0.6605 0.8626 -0.01 ]
###Markdown
다중 배열을 반환하는 유니버설 함수np.modf(arr)는 파이썬 내장함수인 divmod의 벡터화된 버전으로:- arr의 각 원소를 받아, 각 원소의 정수부 배열(np.trunc(arr)과 동일)과- 각 원소의 소수부 배열(arr - np.trunc(arr) 와 동일)을 리턴한다.
###Code
arr = randn(7) * 5
arr
np.modf(arr)
intf = np.trunc(arr)
print arr - intf
print intf
print arr.astype(int)
###Output
[-0.0915 -0.663 0.3731 0.6182 0.45 0.0077 -0.5154]
[-6. -6. 5. 3. 3. 5. -2.]
[-6 -6 5 3 3 5 -2]
###Markdown
4.3 Data processing using arraysnumpy를 사용하면 반복문없이 벡터화된 배열연산으로 순수 python 연산에 비해 수십배 이상 빠르게 데이터를 처리할 수 있다.간단한 예로 $\sqrt{x^2 + y^2}$를 계산하고자 할 때 np.meshgrid를 사용하면:- 동일한 size n의 1d array 두개를 입력받아- 가능한 모든 짝의 (n x n) 2d array를 2개 반환한다.
###Code
points = np.arange(-5, 5, 0.01) # 1000 equally spaced points
print (points[:5]) # fist 5
print (points[-5:]) # last 5
xs, ys = np.meshgrid(points, points)
xs
ys
import matplotlib.pyplot as plt
z = np.sqrt(xs ** 2 + ys ** 2)
z
plt.imshow(z, cmap=plt.cm.gray); plt.colorbar()
plt.title("Image plot of $\sqrt{x^2 + y^2}$ for a grid of values")
plt.draw()
###Output
_____no_output_____
###Markdown
4.3.1 Expressing conditional logic as array operationsnp.where(condition, x, y) 함수는 `x if condition else y`와 같은 3항식의 벡터화된 함수이다.
###Code
xarr = np.array([1.1, 1.2, 1.3, 1.4, 1.5])
yarr = np.array([2.1, 2.2, 2.3, 2.4, 2.5])
cond = np.array([True, False, True, True, False])
result = [(x if c else y)
for x, y, c in zip(xarr, yarr, cond)]
result
###Output
_____no_output_____
###Markdown
위의 방법은 순수 python의 list comprehension을 이용한 반복문으로 배열의 size가 큰 경우에 매우 느리게 동작할 뿐 더러, 배열의 shape이 달라지면(1d array 이상인 경우) 프로그램을 다시 짜야한다.이에 반해 아래와 같이 추상화된 벡터함수 np.where를 사용하면 array에 대한 메모리 블락에 대해 한번에 연산을 처리하여 수백배 이상 더 빠른 속도를 낼 수 있다.
###Code
result = np.where(cond, xarr, yarr)
result
###Output
_____no_output_____
###Markdown
위에서 cond, xarr, yarr의 size는 모두 같았다.그러나, 아래와 같이 xarr나 yarr 둘 중 하나, 또는 모두가 scalar 이어도 broadcasting에 의해 모두 연산 처리된다.
###Code
arr = randn(4, 4)
arr
np.where(arr > 0, 2, -2)
np.where(arr > 0, 2, arr) # set only positive values to 2
###Output
_____no_output_____
###Markdown
np.where를 응용하면, 다음과 같이 두가지의 조건의 조합으로 4가지의 다른 배열 연산을 처리할 수도 있다.- 아래 예는 일종의 함수처럼 활용할 수 있다.- n은 cond1과 cond2의 boolean을 원소로 하는 size n의 1d list/ array이다.순수 python으로 구현한 예:
###Code
# original python list implementation example
cond1 = [True, True, False, False]; cond2 = [True, False, True, False]
result = []
for i in range(len(cond1)):
if cond1[i] and cond2[i]:
result.append(0)
elif cond1[i]:
result.append(1)
elif cond2[i]:
result.append(2)
else:
result.append(3)
result
###Output
_____no_output_____
###Markdown
np.where를 중첩으로 사용하면 위 연산을 :- 훨씬 더 빠른 속도로 동작하면서도 - 더 간결한 코딩으로 구현할 수 있다.numpy array로 구현한 예:
###Code
cond1 = np.array(cond1); cond2 = np.array(cond2)
np.where(cond1 & cond2, 0,
np.where(cond1, 1,
np.where(cond2, 2, 3)))
###Output
_____no_output_____
###Markdown
Boolean type이 산술연산과 만날 때:- True = 1, False= 0으로 형변환 되므로- 아래와 같이 가독성이 떨어지지만, 더 간단한 코딩도 가능하다.
###Code
result = 1 * cond1 + 2 * cond2 - 3 * (cond1 | cond2)
result
result = 3 - (2*cond1 + cond2)
result
###Output
_____no_output_____
###Markdown
4.3.2 Mathematical and statistical methodsndarray는 전체 또는 특정 축에 대한 기본 통계량을 계산하기 위한 메소드를 제공하고 있다. 메소드 설명 sum(axis, dtype, out, keepdims) 주어진 축에 대한 원소의 합을 계산한다. mean(axis, dtype, out, keepdims) 주어진 축에 대한 원소의 평균을 계산한다. std/ var(axis, dtype, out, ddof, keepdims) 주어진 축에 대한 원소의 표준편차/분산을 계산한다. min/ max(axis, out) 주어진 축에 대한 최소/최대 값을 반환한다. argmin/ argmax(axis, out) 주어진 축의 최소/최대 값에 대한 색인을 반환한다. cumsum(axis, dtype, out) 주어진 축에 대한 누적합을 반환한다. cumprod(axis, dtype, out) 주어진 축에 대한 누적곱을 반환한다.
###Code
# arr = np.random.randn(5,4) # normally-distribued data
arr = np.arange(20).reshape((5,4))
print arr
print arr.mean()
print np.mean(arr)
print arr.sum()
###Output
[[ 0 1 2 3]
[ 4 5 6 7]
[ 8 9 10 11]
[12 13 14 15]
[16 17 18 19]]
9.5
9.5
190
###Markdown
mean(axis=n), sum(axis=n) 메소드는 한 차원 낮은 ndarray를 반환한다
###Code
print arr.mean(axis=1)
print arr.sum(0)
###Output
[ 1.5 5.5 9.5 13.5 17.5]
[40 45 50 55]
###Markdown
cumsum(axis=n), cumprod(axis=n) 메소드는 동일한 차원의 연산 중간과정 값을 반환한다.
###Code
arr = np.array([[0, 1, 2], [3, 4, 5], [6, 7, 8]])
print arr.cumsum(0)
print arr.cumprod(1)
###Output
[[ 0 1 2]
[ 3 5 7]
[ 9 12 15]]
[[ 0 0 0]
[ 3 12 60]
[ 6 42 336]]
###Markdown
4.3.3 Methods for boolean arraysboolean의 각 원소의 True는 1로 False는 0으로 산술 연산 처리됨을 확인했다.
###Code
arr = randn(100)
(arr > 0).sum() # Number of positive values
###Output
_____no_output_____
###Markdown
boolean ndarray를 위한 all, any 메소드가 있는 데 이는 특정 축의 각 원소에 대한 `&`, `|` 연산의 집계함수로 이해할 수 있다.- all(axis, out, keepdims): Returns True if all elements evaluate to True.- any(axis, out, keepdims): Returns True if any of the elements of a evaluate to True.
###Code
bools = np.array([False, False, True, False])
bools.any()
bools.all()
###Output
_____no_output_____
###Markdown
4.3.4 Sortingpython의 내장 list처럼 ndarray도 sort 메소드로 객체 자체를 정렬할 수 있다.- sort(axis=-1, kind='quicksort', order=None): Sort an array, in-place.- argsort(axis=-1, kind='quicksort', order=None): Returns the indices that would sort this array.
###Code
arr = randn(8); slic_arr = arr[:2]
arr
arr.sort()
arr
slic_arr
###Output
_____no_output_____
###Markdown
위에서 본 바와 같이 sort 메소드는 ndarray 객체 자체를 변환시킨다.- 이때 새로운 객체를 생성하며, referencing을 달리 하는 것이 아니라- arr 객체 메모리 블럭에 있는 값을 직접적으로 바꾼다.- 아래서 slice View인 slic_arr의 값도 같이 바뀌는 결과가 나타난다.---2차원 이상의 array에서 축을 지정한 sort- axis 속성의 default 값이 -1은 마지막 축을 지정한다.- axis는 크기 비교가 되는 인접 axis의 방향. 또는 순서에 의해 변화될 색인의 축이다.
###Code
arr = randn(5, 3)
arr
arr.sort()
arr
arr.sort(1)
arr
arr.sort(0)
arr
###Output
_____no_output_____
###Markdown
아래는 sort 메소드를 활용하여 분위수를 구하는 예인데:1. arr를 먼저 정렬한다.2. 분위수에 해당하는 특정 위치에 직접 접근한다.
###Code
large_arr = randn(1000)
large_arr.sort()
large_arr[int(0.05 * len(large_arr))] # 5% quantile
###Output
_____no_output_____
###Markdown
argsort이번에는 정렬된 index를 반환하는 argsort 메소드를 보자. arr = np.array([[1,6,2], [5,0,1], [4,3,7]])arr 아래와 같이, axis index에 따라 정렬한 후 순서에 대한 색인을 값으로 같는다.
###Code
sort1i = arr.argsort(axis=1)
sort1i
arr[0][sort1i[0]]
arr.argsort(axis=0)
###Output
_____no_output_____
###Markdown
참고로 numpy.sort(arr, axis=-1, kind='quicksort', order=None) 함수는 - arr 자체를 변환시키지 않고, - 정렬된 arr의 copy를 반환한다.반면 arr 자체를 반환시키지 않는 argsort메소드와 np.argsort 함수는 동일하다.---order 옵션은 field name 또는 colname이 주어진 경우 활용하는 데:- numpy에서 다루기 보다는 pandas나 scikit-learn에서 다루는 것이 적절하다.- 보통 dtype과 함께 사용하며, 여기서 간단히 소개하면,- 지정된 field를 기준으로 정렬을 실시한다.
###Code
dtype = [('name', 'S10'), ('height', float), ('age', int)]
values = [('Arthur', 1.8, 41), ('Lancelot', 1.9, 38),
('Galahad', 1.7, 38)]
a = np.array(values, dtype=dtype)
a
np.sort(a, order='height')
a.shape
a.dtype
a.data
###Output
_____no_output_____
###Markdown
4.3.5 Unique and other set logicnumpy는 1d array를 위한 몇가지 집합연산을 위한 함수를 제공한다.numpy.unique(arr, return_index=False, return_inverse=False, return_counts=False, axis=None):- 입력 배열에서 유일한 원소를 정렬하여 반환하며, 옵션에 따라 3가지의 배열을 더 반환할 수 있다.- arr: 유일한 원소를 뽑아낼 입력 배열- return_index: 출력 배열을 생산할 수 있는 입력 arr의 index 배열 - 축이 지정되지 않는 경우(None), flattend 1d array를 출력한다.- return_inverse: 입력 arr를 재생산할 수 있는 출력 arr의 index 배열- return_counts: 각 유일 원소의 arr에서의 출현 빈도를 나타내는 배열numpy.intersect1d(ar1, ar2, assume_unique=False):- 두 1d array의 교집합을 리턴- assume_unique: 두 입력 arr가 모두 set이라는 가정. 속도를 증가시킨다.numpy.union1d(ar1, ar2):- 두 입력 1d array의 합집합을 정렬하여 반환numpy.in1d(ar1, ar2, assume_unique=False, invert=False):- ar1원소가 ar2원소를 포함되는 지 여부를 boolean array로 반환. size는 ar1과 동일.- invert: ar2원소가 ar1원소에 포함되는 지 여부를 boolean array로 반환. size는 ar2와 동일. - numpy.in1d(ar1, ar2, invert=True)는 numpy.in1d(ar2, ar1)과 동일. numpy.isin(element, test_elements, assume_unique=False, invert=False):- element의 각 원소가 flatten된 test_elements에 포함되는 지 여부를 반환. shape은 elemnet와 동일- in1d의 n 차원으로 확장한 함수numpy.setdiff1d(ar1, ar2, assume_unique=False);- ar1 - ar2의 차집합을 정렬하여 반환한다.numpy.setxor1d(ar1, ar2, assume_unique=False):- 두 배열의 합집합에서 교집합을 뺀 배열을 정렬하여 반환
###Code
names = np.array(['Bob', 'Joe', 'Will', 'Bob', 'Will', 'Joe', 'Joe'])
np.unique(names)
ints = np.array([3, 3, 3, 2, 2, 1, 1, 4, 4])
np.unique(ints)
sorted(set(names))
values = np.array([6, 0, 0, 3, 2, 5, 6])
np.in1d(values, [2, 3, 6])
###Output
_____no_output_____
###Markdown
4.4. File input and output with arrays텍스트나 바이너리 데이터를 파일에서 읽거나 쓸 수 있다. 4.4.1 Storing arrays on disk in binary formatnp.save와 np.load는 압축되지 않는 raw 바이너리 형식으로 .npy 파일에서 ndarray 객체를 저장하거나 읽는다.- 기본적으로 pickle을 사용하며, python 2와 3에서 모두 호환되도록 저장한다.- pickle의 dump와 load를 사용하는 것이 일반적이므로, 굳이 알 필요 없다.
###Code
arr = np.arange(10)
np.save('some_array', arr)
%ls
np.load('some_array.npy')
###Output
_____no_output_____
###Markdown
np.savez 함수를 사용하면, 여러 ndarray를 압축된 형식으로 저장한다.- 저장하려는 배열은 키워드 인자와 함께 저장하여,- np.load로 불러올 때 사전 형식으로 객체를 호출하 수 잇다.
###Code
np.savez('array_archive.npz', a=arr, b=arr)
arch = np.load('array_archive.npz')
arch['b']
# !rm some_array.npy
# !rm array_archive.npz
del arch
!del some_array.npy
!del array_archive.npz
###Output
_____no_output_____
###Markdown
4.4.2 Saving and loading text files보통은 pandas의 read_csv 또는 read_table로 텍스트 파일을 읽어 오겠지만, np.loadtxt나 np.getfromtxt를 이용할 경우도 있다.np.loadtxt는 구분자 지정, 특정 컬럼 변환함수 지정, row skip 등의 기능을 제공한다.
###Code
!type ..\examples\array_ex.txt
arr = np.loadtxt('../examples/array_ex.txt', delimiter=',')
arr
###Output
_____no_output_____
###Markdown
np.savetxt는 배열을 텍스트 파일로 저장한다.getfromtxt는 loadtxt와 유사하지만, 구조화된 배열과 누락된 데이터 처리를 위해 설계되었다. 4.5 Linear algebranumpy는 다양한 행렬 연산을 지원하고 있어, 대학 선형대수를 위한 python 교재도 있다.- 기계학습/ deep learning을 위한 새로운 알로리즘 개발을 위해서는 중요한 내용이다. numpy 함수 설명 diag(a) 정방행렬의 대각요소를 반환하거나, 1d 행렬을 대각화 변환 dot(a) 행렬 곱 계산 trace(a) 행렬 trace(대각요소의 합)을 계산 linalg.det(a) a의 determinet 계산 linalg.eig(a) 정방행렬 a에 대한 고유치와 고유벡터를 계산 linalg.inv(a) a의 역행렬 계산 linalg.qr(a) qr 분해를 계산 linalg.svd(a) Singular Value Decomposition linalg.solve(a, b) ax = b의 행렬방적의 해 linalg.lstsq(X, y) y = wx를 만족하는 최소제곱 해(w)를 구한다.
###Code
x = np.array([[1., 2., 3.], [4., 5., 6.]])
y = np.array([[6., 23.], [-1, 7], [8, 9]])
print x
print y
x.dot(y) # equivalently np.dot(x, y)
np.dot(x, np.ones(3))
try: np.dot(x, y.T)
except ValueError: print "you should match the shapes of two matrix"
###Output
you should match the shapes of two matrix
###Markdown
numpy.linalg에는 행력의 분할과 역행렬, 행렬식 같은 것을 포함한다.
###Code
np.random.seed(12345)
from numpy.linalg import inv, qr
X = randn(5, 5)
mat = X.T.dot(X)
mat
inv(mat)
mat.dot(inv(mat))
###Output
_____no_output_____
###Markdown
아래 예제는 선형회귀 등에서 최소제곱법 등에 활용한 QR 분해를 구하는 방법을 나타냈다.- QR 분해의 자세한 내용은 [위키백과](https://ko.wikipedia.org/wiki/QR_%EB%B6%84%ED%95%B4)를 참고
###Code
q, r = qr(mat)
r
###Output
_____no_output_____
###Markdown
4.6 Random number generation다양한 분포의 random 함수를 제공한다.아래에서 보면 random.normal과 random.randn은 같은 결과를 제공한다.
###Code
np.random.seed(12345)
samples = np.random.normal(size=(4, 4))
np.random.seed(12345)
samples2 = randn(4,4)
print samples
print samples2
###Output
[[-0.2047 0.4789 -0.5194 -0.5557]
[ 1.9658 1.3934 0.0929 0.2817]
[ 0.769 1.2464 1.0072 -1.2962]
[ 0.275 0.2289 1.3529 0.8864]]
[[-0.2047 0.4789 -0.5194 -0.5557]
[ 1.9658 1.3934 0.0929 0.2817]
[ 0.769 1.2464 1.0072 -1.2962]
[ 0.275 0.2289 1.3529 0.8864]]
###Markdown
아래 예에서 보듯이 numpy.random 모듈은 python 내장 random 모듈에 비해 훨씬 더 빠른 sample 추출이 가능하다.- normalvariate(mu, gamma): 정규분포로 부터 하나의 sample을 추출한다.
###Code
from random import normalvariate
N = 1000000
%timeit samples = [normalvariate(0, 1) for _ in xrange(N)]
%timeit np.random.normal(size=N)
###Output
1 loop, best of 3: 761 ms per loop
10 loops, best of 3: 36.1 ms per loop
###Markdown
numpy.random 모듈에 대한 자세한 설명은 pandas에서 정리할 계획이다.일부 numpy.random 함수: 함수 설명 seed 난수 발생 seed를 지정 permutation 순서를 임의로 바꾸거나 임의의 순열을 반환 shuffle 리스트나 배열의 순서를 뒤섞는다. rand 균등 분포에서 표본을 추출 randint 주어진 최소/최대 범위에서 임의 난수 추출 randn 표준정규분포에서 표본 추출 binomial 이항분포에서 표본 추출 normal 정규분포에서 표본 추출 beta 베타분포에서 표본 추출 chisquare 카이스퀘어 분포에서 표본 추출 gamma 감마분포에서 표본 추출 4.7 Example: Random Walks무작위 계단오르내리기 예제로서, 순서 python으로 작업하면 아래와 같다.
###Code
import random
position = 0
walk = [position]
steps = 1000
for i in xrange(steps):
step = 1 if random.randint(0, 1) else -1
position += step
walk.append(position)
print position
np.random.seed(12345)
nsteps = 1000
draws = np.random.randint(0, 2, size=nsteps)
steps = np.where(draws > 0, 1, -1)
walk = steps.cumsum()
walk[-1]
walk.min()
walk.max()
###Output
_____no_output_____
###Markdown
최초로 10 계단 이상 오르내린 시행은 어디일까?
###Code
(np.abs(walk) >= 10).argmax()
walk[37]
###Output
_____no_output_____
###Markdown
4.7.1 Simulating many random walks at once대량의 random walk 실험을 한번에 빠르게 수행하는 손쉬운 방법이 있다.- random walk를 수행하는 2d ndarray를 생성하고, 누적합을 구하면 된다.
###Code
nwalks = 5000
nsteps = 1000
draws = np.random.randint(0, 2, size=(nwalks, nsteps)) # 0 or 1
steps = np.where(draws > 0, 1, -1)
walks = steps.cumsum(1)
walks
print walks.max()
print walks.min()
hits30 = (np.abs(walks) >= 30).any(1)
hits30
hits30.sum() # Number that hit 30 or -30
###Output
_____no_output_____
###Markdown
최초 30 계단을 오르내린 시점은 언제인가?
###Code
crossing_times = (np.abs(walks[hits30]) >= 30).argmax(1)
crossing_times.mean()
###Output
_____no_output_____
###Markdown
다음과 같이 다양한 분포로부터 random walk를 생성할 수 있다.
###Code
steps = np.random.normal(loc=0, scale=0.25,
size=(nwalks, nsteps))
###Output
_____no_output_____ |
week12/oil-production-sklearn.ipynb | ###Markdown
This example is based on the multiple regression example in Table 14.6 from Ken Black (2010). *Business Statistics for Contemporary Decision Making*, 6th Edition, John Wiley and Sons. p. 567.Import dependent libraries: pandas (`pd`) for data manipulation, sklearn's `linear_model` for statistics, and matplotlib's pyplot (`plt`) for visualization.
###Code
import pandas as pd
from sklearn import linear_model
import matplotlib.pyplot as plt
###Output
_____no_output_____
###Markdown
First, make sure that the data file `data-oil-production.csv` is accessible to your Python environment. If you are using your local machine, you can put it in the same directory as this Jupyter notebook. If you are using a cloud service like Google Colab, make sure to upload the file to the remote machine (expand folder icon on left side and click upload button).Next, read the data file using pandas.
###Code
df = pd.read_csv('data-oil-production.csv')
display(df)
###Output
_____no_output_____
###Markdown
Separate the dependent variable (`y`) from the independent variables (`X`).
###Code
y = df.CrudeProduction
X = df[['EnergyConsumption', 'NuclearElectricity', 'CoalProduction', 'GasProduction', 'AutoMPG']]
###Output
_____no_output_____
###Markdown
Build and fit a linear model using the independent and dependent variables.
###Code
model = linear_model.LinearRegression().fit(X, y)
print('intercept = {:}'.format(model.intercept_))
print('coef = {:}'.format(model.coef_))
print('R^2 = {:.3f}'.format(model.score(X,y)))
y_hat = model.predict(X)
plt.figure()
plt.scatter(y_hat, y-y_hat)
plt.xlabel('Predicted Crude Production')
plt.ylabel('Residuals')
plt.show()
###Output
intercept = 2.708473950357117
coef = [ 0.83567046 -0.00654432 0.00982508 -0.14321095 -0.73414356]
R^2 = 0.921
###Markdown
A LASSO procedure can also be used to select features.
###Code
model = linear_model.Lasso(alpha=1).fit(X, y)
print('coef = {:}'.format(model.coef_))
print('intercept = {:}'.format(model.intercept_))
print('R^2 = {:.3f}'.format(model.score(X,y)))
y_hat = model.predict(X)
plt.figure()
plt.scatter(y_hat, y-y_hat)
plt.xlabel('Predicted Crude Production')
plt.ylabel('Residuals')
plt.show()
###Output
coef = [ 0.71961883 -0.00800783 0.00244795 0. -0. ]
intercept = 3.258178633036067
R^2 = 0.900
|
Estudos/Corretor_Ortografico_NLP/Corretor.ipynb | ###Markdown
Gerando Palavras através de Tokens
###Code
palavras_separadas = nltk.tokenize.word_tokenize(artigos)
len(palavras_separadas)
###Output
_____no_output_____
###Markdown
Separa as palavras e adiciona a uma lista
###Code
def separa_palavras(lista_tokens):
lista_palavras = []
for token in lista_tokens:
if token.isalpha():
lista_palavras.append(token)
return lista_palavras
lista_palavras = separa_palavras(palavras_separadas)
print(lista_palavras[:10])
print(f'O número de palavras é {len(lista_palavras)}')
###Output
O número de palavras é 403106
###Markdown
Normaliza palavras em minúsculas
###Code
def normalizacao(lista_palavras):
lista_normalizada = []
for palavra in lista_palavras:
lista_normalizada.append(palavra.lower())
return lista_normalizada
lista_normalizada = normalizacao(lista_palavras)
print(lista_normalizada[:10])
###Output
['imagem', 'temos', 'a', 'seguinte', 'classe', 'que', 'representa', 'um', 'usuário', 'no']
###Markdown
Variáveis
###Code
palavra_ex = 'lgica'
frequencia = nltk.FreqDist(lista_normalizada)
total_palavras = len(lista_normalizada)
###Output
_____no_output_____
###Markdown
Função que Insere letras
###Code
def insere_letras(fatias):
novas_palavras = []
letras = 'abcdefghijklmnopqrstuvwxyzáâàãäéêèëíîìïóôòöõúûùüç'
for E, D in fatias:
for letra in letras:
novas_palavras.append(E + letra + D)
return novas_palavras
###Output
_____no_output_____
###Markdown
Função Corretor e Função Probabilidade
###Code
def corretor(palavra):
palavras_geradas = gerador_palavras(palavra)
palavra_correta = max(palavras_geradas, key=probabilidade)
return palavra_correta
def probabilidade(palavra_gerada):
return frequencia[palavra_gerada] / total_palavras
###Output
_____no_output_____
###Markdown
Gerador de palavras Fatiadas
###Code
def gerador_palavras(palavra):
fatias = []
for i in range(len(palavra)+1):
fatias.append((palavra[:i], palavra[i:]))
palavras_geradas = insere_letras(fatias)
return palavras_geradas
palavras_geradas = gerador_palavras(palavra_ex)
print(palavras_geradas)
corretor('lgica')
###Output
_____no_output_____
###Markdown
Função que Gera dados para teste
###Code
def cria_dados_teste(nome_arquivo):
lista_palavras_teste = []
f = open(nome_arquivo, 'r', encoding="utf8")
for linha in f:
correta, errada = linha.split()
lista_palavras_teste.append((correta, errada))
f.close()
return lista_palavras_teste
lista_teste = cria_dados_teste('dados/palavras.txt')
lista_teste
###Output
_____no_output_____
###Markdown
função Avaliadora dos testes
###Code
def avaliador(testes):
numero_palavras = len(testes)
acertou = 0
for correta, errada in testes:
palavra_corrigida = corretor(errada)
if palavra_corrigida == correta:
acertou += 1
taxa_acerto = (acertou/numero_palavras)*100
print('{} palavras analisadas.. Taxa de acerto de {:.2f}%'.format(numero_palavras, taxa_acerto))
avaliador(lista_teste)
###Output
186 palavras analisadas.. Taxa de acerto de 1.08%
###Markdown
Função que Deleta caracteres
###Code
def deletando_caracters(fatias):
novas_palavras = []
for E, D in fatias:
novas_palavras.append(E + D[1:])
return novas_palavras
###Output
_____no_output_____
###Markdown
Gerador de palavras Fatiadas 2.0
###Code
def gerador_palavras(palavra):
fatias = []
for i in range(len(palavra)+1):
fatias.append((palavra[:i], palavra[i:]))
palavras_geradas = insere_letras(fatias)
palavras_geradas += deletando_caracters(fatias)
return palavras_geradas
palavras_geradas = gerador_palavras(palavra_ex)
print(palavras_geradas)
avaliador(lista_teste)
###Output
186 palavras analisadas.. Taxa de acerto de 41.40%
###Markdown
Função Troca Letras
###Code
def troca_letra(fatias):
novas_palavras = []
letras = 'abcdefghijklmnopqrstuvwxyzáâàãäéêèëíîìïóôòöõúûùüç'
for E, D in fatias:
for letra in letras:
novas_palavras.append(E + letra + D[1:])
return novas_palavras
###Output
_____no_output_____
###Markdown
Gerador de palavras 3.0
###Code
def gerador_palavras(palavra):
fatias = []
for i in range(len(palavra)+1):
fatias.append((palavra[:i], palavra[i:]))
palavras_geradas = insere_letras(fatias)
palavras_geradas += deletando_caracters(fatias)
palavras_geradas += troca_letra(fatias)
return palavras_geradas
palavras_geradas = gerador_palavras(palavra_ex)
print(palavras_geradas)
avaliador(lista_teste)
###Output
186 palavras analisadas.. Taxa de acerto de 76.34%
###Markdown
Função Inverte Letras
###Code
def inverte_letras(fatias):
novas_palavras = []
for E, D in fatias:
if len(D) > 1:
novas_palavras.append(E + D[1] + D[0] + D[2:])
return novas_palavras
###Output
_____no_output_____
###Markdown
Gerador de palavras 4.0
###Code
def gerador_palavras(palavra):
fatias = []
for i in range(len(palavra)+1):
fatias.append((palavra[:i], palavra[i:]))
palavras_geradas = insere_letras(fatias)
palavras_geradas += deletando_caracters(fatias)
palavras_geradas += troca_letra(fatias)
palavras_geradas += inverte_letras(fatias)
return palavras_geradas
palavras_geradas = gerador_palavras(palavra_ex)
print(palavras_geradas)
avaliador(lista_teste)
###Output
186 palavras analisadas.. Taxa de acerto de 76.34%
###Markdown
Novo Avaliador
###Code
def avaliador(testes, vocabulario):
numero_palavras = len(testes)
acertou = 0
desconhecida = 0
for correta, errada in testes:
palavra_corrigida = corretor(errada)
if palavra_corrigida == correta:
acertou += 1
else:
desconhecida += (correta not in vocabulario)
taxa_acerto = (acertou/numero_palavras)*100
taxa_desconhecida = (desconhecida/numero_palavras)*100
print('{} palavras analisadas.. Taxa de acerto de {:.2f}%, desconhecida é {:.2f}%'.format(numero_palavras, taxa_acerto, taxa_desconhecida))
vocabulario = set(lista_normalizada)
avaliador(lista_teste, vocabulario)
###Output
186 palavras analisadas.. Taxa de acerto de 76.34%, desconhecida é 6.99%
|
FineTuning_BERT/DistilBERT_SST2_FineTuning_HF.ipynb | ###Markdown
Fine Tuning the Pretrained DistilBert on Yelp Review Dataset for Sentiment Prediction
###Code
gpu_info = !nvidia-smi
gpu_info = '\n'.join(gpu_info)
if gpu_info.find('failed') >= 0:
print('Not connected to a GPU')
else:
print(gpu_info)
import warnings
warnings.filterwarnings('ignore')
import pandas as pd
import numpy as np
from sklearn.model_selection import train_test_split
import transformers
import tensorflow as tf
import datasets
from datasets import Dataset, load_dataset
from transformers import AutoTokenizer
from transformers import TFAutoModelForSequenceClassification
from transformers import pipeline
from datasets import load_metric
df = pd.read_csv("yelp.csv")
df_bert = df[["text", "stars"]]
###Output
_____no_output_____
###Markdown
Preprocess
###Code
df_bert["stars"] = df_bert["stars"].apply(lambda x:1 if x in [4,5] else (0 if x in [1,2] else 3))
df_bert.drop(df_bert[df_bert.stars == 3].index, inplace = True)
df_bert
df_bert.shape
train, eval = train_test_split(df_bert,
test_size = 0.2,
random_state = 123)
###Output
_____no_output_____
###Markdown
------------------------------------------------------------------------------------------------------------------------------
###Code
# export train, valid, test in csv format
train.to_csv("/Users/alex/Desktop/github_repo/NLP/finetuning_bert/train_ft.csv", index=False, header = True)
valid.to_csv("/Users/alex/Desktop/github_repo/NLP/finetuning_bert/valid_ft.csv", index=False, header = True)
test.to_csv("/Users/alex/Desktop/github_repo/NLP/finetuning_bert/test_ft.csv", index=False, header = True)
# Load train, valid, test csv datasets converting them into datasets.dataDict format for Arrow
dataset = load_dataset('csv', data_files={'train': 'train_ft.csv',
'valid':'valid_ft.csv',
'test':'test_ft.csv'})
###Output
Using custom data configuration default-5945906af8db4695
###Markdown
------------------------------------------------------------------------------------------------------------------------------
###Code
# convert train, valid, test datasets into dataDict format for the use of Arrow
train_ = Dataset.from_pandas(train)
eval_ = Dataset.from_pandas(eval)
# train test split (test = set for validation)
train_ = train_.train_test_split(test_size = 0.2)
train_
# tokenizer for the pretrained distilbert
tokenizer = AutoTokenizer.from_pretrained("distilbert-base-uncased-finetuned-sst-2-english")
# Tokenize all the dataDicts padding and truncating the texts
def tokenize_function(dataset):
return tokenizer(dataset["text"], padding="max_length", truncation=True)
train_token = train_['train'].map(tokenize_function, batched=True)
test_token = train_['test'].map(tokenize_function, batched=True)
eval_token = eval_.map(tokenize_function, batched=True)
# Use only subset of the datasets
train_sub = train_token.shuffle(seed=123).select(range(500))
test_sub = test_token.shuffle(seed=123).select(range(50))
eval_sub = eval_token.shuffle(seed=123).select(range(50))
# Load the pretrained distilBert model
model = TFAutoModelForSequenceClassification.from_pretrained("distilbert-base-uncased-finetuned-sst-2-english", num_labels=2)
# Set the datasets in tensorflow format
train_tf = train_sub.remove_columns(["text"]).with_format("tensorflow")
test_tf = test_sub.remove_columns(["text"]).with_format("tensorflow")
eval_tf = eval_sub.remove_columns(["text"]).with_format("tensorflow")
train_tf
# convert everything in big tensor
# batch_size = 8
train_features = {x: train_tf[x] for x in ['input_ids','attention_mask']}
train_tf_dataset = tf.data.Dataset.from_tensor_slices((train_features, train_tf["stars"]))
train_tf_dataset = train_tf_dataset.shuffle(len(train_tf)).batch(8)
test_features = {x: test_tf[x] for x in ['input_ids','attention_mask']}
test_tf_dataset = tf.data.Dataset.from_tensor_slices((test_features, test_tf["stars"]))
test_tf_dataset = test_tf_dataset.batch(8)
eval_features = {x: eval_tf[x] for x in ['input_ids','attention_mask']}
eval_tf_dataset = tf.data.Dataset.from_tensor_slices((eval_features, eval_tf["stars"]))
eval_tf_dataset = eval_tf_dataset.batch(8)
from tensorflow.keras.optimizers.schedules import PolynomialDecay
batch_size = 8
num_epochs = 3
# The number of training steps is the number of samples in the dataset, divided by the batch size then multiplied
# by the total number of epochs
num_train_steps = len(train_tf_dataset) * num_epochs
lr_scheduler = PolynomialDecay(
initial_learning_rate=5e-5, end_learning_rate=0.0, decay_steps=num_train_steps
)
from tensorflow.keras.optimizers import Adam
optimizer = Adam(learning_rate=lr_scheduler)
# Compile and train the model with keras
# model = distilbert-base-cased
model.compile(
optimizer=optimizer,
loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True),
metrics=tf.metrics.SparseCategoricalAccuracy(),
)
model.fit(train_tf_dataset, validation_data=eval_tf_dataset, epochs=num_epochs)
# Evaluate on eval_tf_dataset
pred = model.predict(test_tf_dataset)["logits"]
class_pred = np.argmax(pred, axis=1)
print(pred.shape, class_pred.shape)
metric = load_metric("glue", "mrpc")
metric.compute(predictions = class_pred, references = test_sub["stars"])
classifier = pipeline('sentiment-analysis', model=model, tokenizer=tokenizer)
classifier('it is too bad')
###Output
_____no_output_____ |
2_TCN/TCN_TREC.ipynb | ###Markdown
TCN Classification with MR DatasetWe will build a text classification model using TCN model on the Movie Review Dataset. Since there is no standard train/test split for this dataset, we will use 10-Fold Cross Validation (CV). Load the library
###Code
import tensorflow as tf
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
import seaborn as sns
import re
import nltk
import random
# from nltk.tokenize import TweetTokenizer
from sklearn.model_selection import KFold
%config IPCompleter.greedy=True
%config IPCompleter.use_jedi=False
# nltk.download('twitter_samples')
tf.config.list_physical_devices('GPU')
###Output
_____no_output_____
###Markdown
Load the Dataset
###Code
corpus = pd.read_pickle('../../../0_data/TREC/TREC.pkl')
corpus.label = corpus.label.astype(int)
print(corpus.shape)
corpus
corpus.info()
corpus.groupby(by=['split', 'label']).count()
# Separate the sentences and the labels
# Separate the sentences and the labels for training and testing
train_x = list(corpus[corpus.split=='train'].sentence)
train_y = np.array(corpus[corpus.split=='train'].label)
print(len(train_x))
print(len(train_y))
test_x = list(corpus[corpus.split=='test'].sentence)
test_y = np.array(corpus[corpus.split=='test'].label)
print(len(test_x))
print(len(test_y))
train_x[0]
###Output
_____no_output_____
###Markdown
Data PreprocessingPreparing data for word embedding, especially for pre-trained word embedding like Word2Vec or GloVe, __don't use standard preprocessing steps like stemming or stopword removal__. Compared to our approach on cleaning the text when doing word count based feature extraction (e.g. TFIDF) such as removing stopwords, stemming etc, now we will keep these words as we do not want to lose such information that might help the model learn better.__Tomas Mikolov__, one of the developers of Word2Vec, in _word2vec-toolkit: google groups thread., 2015_, suggests only very minimal text cleaning is required when learning a word embedding model. Sometimes, it's good to disconnectIn short, what we will do is:- Puntuations removal- Lower the letter case- TokenizationThe process above will be handled by __Tokenizer__ class in TensorFlow- One way to choose the maximum sequence length is to just pick the length of the longest sentence in the training set.
###Code
# Define a function to compute the max length of sequence
def max_length(sequences):
'''
input:
sequences: a 2D list of integer sequences
output:
max_length: the max length of the sequences
'''
max_length = 0
for i, seq in enumerate(sequences):
length = len(seq)
if max_length < length:
max_length = length
return max_length
from tensorflow.keras.preprocessing.text import Tokenizer
from tensorflow.keras.preprocessing.sequence import pad_sequences
trunc_type='post'
padding_type='post'
oov_tok = "<UNK>"
# Separate the sentences and the labels
train_x = list(corpus[corpus.split=='train'].sentence)
train_y = np.array(corpus[corpus.split=='train'].label)
test_x = list(corpus[corpus.split=='test'].sentence)
test_y = np.array(corpus[corpus.split=='test'].label)
# Cleaning and Tokenization
tokenizer = Tokenizer(oov_token=oov_tok)
tokenizer.fit_on_texts(train_x)
print("Example of sentence: ", train_x[4])
# Turn the text into sequence
training_sequences = tokenizer.texts_to_sequences(train_x)
max_len = max_length(training_sequences)
print('Into a sequence of int:', training_sequences[4])
# Pad the sequence to have the same size
training_padded = pad_sequences(training_sequences, maxlen=max_len, padding=padding_type, truncating=trunc_type)
print('Into a padded sequence:', training_padded[4])
# See the first 10 words in the vocabulary
word_index = tokenizer.word_index
for i, word in enumerate(word_index):
print(word, word_index.get(word))
if i==9:
break
vocab_size = len(word_index)+1
print(vocab_size)
###Output
<UNK> 1
the 2
what 3
is 4
of 5
in 6
a 7
how 8
's 9
was 10
8461
###Markdown
Model 1: Embedding Random TCN ModelNow, we will build Temporal Convolutional Network (CNN) models to classify encoded documents as either positive or negative.The model takes inspiration from https://github.com/philipperemy/keras-tcn and https://www.kaggle.com/christofhenkel/temporal-convolutional-network__Arguments__`TCN(nb_filters=64, kernel_size=2, nb_stacks=1, dilations=[1, 2, 4, 8, 16, 32], padding='causal', use_skip_connections=False, dropout_rate=0.0, return_sequences=True, activation='relu', kernel_initializer='he_normal', use_batch_norm=False, **kwargs)`- `nb_filters`: Integer. The number of filters to use in the convolutional layers. Would be similar to units for LSTM.- `kernel_size`: Integer. The size of the kernel to use in each convolutional layer.- `dilations`: List. A dilation list. Example is: [1, 2, 4, 8, 16, 32, 64].- `nb_stacks`: Integer. The number of stacks of residual blocks to use.- `padding`: String. The padding to use in the convolutions. 'causal' for a causal network (as in the original implementation) and - `'same' for a non-causal network.- `use_skip_connections`: Boolean. If we want to add skip connections from input to each residual block.- `return_sequences`: Boolean. Whether to return the last output in the output sequence, or the full sequence.- `dropout_rate`: Float between 0 and 1. Fraction of the input units to drop.- `activation`: The activation used in the residual blocks o = activation(x + F(x)).- `kernel_initializer`: Initializer for the kernel weights matrix (Conv1D).- `use_batch_norm`: Whether to use batch normalization in the residual layers or not.- `kwargs`: Any other arguments for configuring parent class Layer. For example "name=str", Name of the model. Use unique names when using multiple TCN. Now, we will define our TCN model as follows:- One TCN layer with 100 filters, kernel size 1-6, and relu and tanh activation function;- Dropout size = 0.5;- Optimizer: Adam (The best learning algorithm so far)- Loss function: binary cross-entropy (suited for binary classification problem)**Note**: - The whole purpose of dropout layers is to tackle the problem of over-fitting and to introduce generalization to the model. Hence it is advisable to keep dropout parameter near 0.5 in hidden layers. - https://missinglink.ai/guides/keras/keras-conv1d-working-1d-convolutional-neural-networks-keras/
###Code
from tcn import TCN, tcn_full_summary
from tensorflow.keras.layers import Input, Embedding, Dense, Dropout, SpatialDropout1D
from tensorflow.keras.layers import concatenate, GlobalAveragePooling1D, GlobalMaxPooling1D
from tensorflow.keras.models import Model
def define_model(kernel_size = 3, activation='relu', input_dim = None, output_dim=300, max_length = None ):
inp = Input( shape=(max_length,))
x = Embedding(input_dim=input_dim, output_dim=output_dim, input_length=max_length)(inp)
x = SpatialDropout1D(0.1)(x)
x = TCN(128,dilations = [1, 2, 4], return_sequences=True, activation = activation, name = 'tcn1')(x)
x = TCN(64,dilations = [1, 2, 4], return_sequences=True, activation = activation, name = 'tcn2')(x)
avg_pool = GlobalAveragePooling1D()(x)
max_pool = GlobalMaxPooling1D()(x)
conc = concatenate([avg_pool, max_pool])
conc = Dense(16, activation="relu")(conc)
conc = Dropout(0.1)(conc)
outp = Dense(6, activation="softmax")(conc)
model = Model(inputs=inp, outputs=outp)
model.compile( loss = 'sparse_categorical_crossentropy', optimizer = 'adam', metrics = ['accuracy'])
return model
model_0 = define_model( input_dim=1000, max_length=100)
model_0.summary()
# tcn_full_summary(model_0)
# class myCallback(tf.keras.callbacks.Callback):
# # Overide the method on_epoch_end() for our benefit
# def on_epoch_end(self, epoch, logs={}):
# if (logs.get('accuracy') > 0.93):
# print("\nReached 93% accuracy so cancelling training!")
# self.model.stop_training=True
callbacks = tf.keras.callbacks.EarlyStopping(monitor='val_accuracy', min_delta=0,
patience=20, verbose=2,
mode='auto', restore_best_weights=True)
###Output
_____no_output_____
###Markdown
Train and Test the Model
###Code
# Parameter Initialization
trunc_type='post'
padding_type='post'
oov_tok = "<UNK>"
activations = ['relu', 'tanh']
filters = 100
kernel_sizes = [1, 2, 3, 4, 5, 6]
columns = ['Activation', 'Filters', 'Acc']
record = pd.DataFrame(columns = columns)
# Separate the sentences and the labels
train_x = list(corpus[corpus.split=='train'].sentence)
train_y = np.array(corpus[corpus.split=='train'].label)
test_x = list(corpus[corpus.split=='test'].sentence)
test_y = np.array(corpus[corpus.split=='test'].label)
exp = 0
for activation in activations:
for kernel_size in kernel_sizes:
exp+=1
print('-------------------------------------------')
print('Training {}: {} activation, {} kernel size.'.format(exp, activation, kernel_size))
print('-------------------------------------------')
# encode data using
# Cleaning and Tokenization
tokenizer = Tokenizer(oov_token=oov_tok)
tokenizer.fit_on_texts(train_x)
# Turn the text into sequence
training_sequences = tokenizer.texts_to_sequences(train_x)
test_sequences = tokenizer.texts_to_sequences(test_x)
max_len = max_length(training_sequences)
# Pad the sequence to have the same size
Xtrain = pad_sequences(training_sequences, maxlen=max_len, padding=padding_type, truncating=trunc_type)
Xtest = pad_sequences(test_sequences, maxlen=max_len, padding=padding_type, truncating=trunc_type)
word_index = tokenizer.word_index
vocab_size = len(word_index)+1
# Define the input shape
model = define_model(kernel_size, activation, input_dim=vocab_size, max_length=max_len)
# Train the model and initialize test accuracy with 0
acc = 0
while(acc<0.7):
model.fit(Xtrain, train_y, batch_size=50, epochs=100, verbose=1,
callbacks=[callbacks], validation_data=(Xtest, test_y))
# evaluate the model
loss, acc = model.evaluate(Xtest, test_y, verbose=0)
print('Test Accuracy: {}'.format(acc*100))
if (acc<0.6):
print('The model suffered from local minimum. Retrain the model!')
model = define_model(kernel_size, activation, input_dim=vocab_size, max_length=max_len)
else:
print('Done!')
parameters = [activation, kernel_size]
entries = parameters + [acc]
temp = pd.DataFrame([entries], columns=columns)
record = record.append(temp, ignore_index=True)
print()
print(record)
print()
###Output
-------------------------------------------
Training 1: relu activation, 1 kernel size.
-------------------------------------------
Epoch 1/100
110/110 [==============================] - 24s 139ms/step - loss: 1.5604 - accuracy: 0.3325 - val_loss: 0.8038 - val_accuracy: 0.7200
Epoch 2/100
110/110 [==============================] - 10s 94ms/step - loss: 0.6618 - accuracy: 0.7846 - val_loss: 0.5389 - val_accuracy: 0.8120
Epoch 3/100
110/110 [==============================] - 10s 93ms/step - loss: 0.3117 - accuracy: 0.9115 - val_loss: 0.4700 - val_accuracy: 0.8760
Epoch 4/100
110/110 [==============================] - 10s 94ms/step - loss: 0.1802 - accuracy: 0.9460 - val_loss: 0.5101 - val_accuracy: 0.8640
Epoch 5/100
110/110 [==============================] - 10s 95ms/step - loss: 0.1116 - accuracy: 0.9617 - val_loss: 0.5702 - val_accuracy: 0.8620 0.1115 - accuracy
Epoch 6/100
110/110 [==============================] - 10s 95ms/step - loss: 0.0821 - accuracy: 0.9690 - val_loss: 0.5980 - val_accuracy: 0.8420
Epoch 7/100
110/110 [==============================] - 10s 94ms/step - loss: 0.2799 - accuracy: 0.9056 - val_loss: 0.8327 - val_accuracy: 0.8580
Epoch 8/100
110/110 [==============================] - 11s 98ms/step - loss: 0.0579 - accuracy: 0.9817 - val_loss: 0.6182 - val_accuracy: 0.8820
Epoch 9/100
110/110 [==============================] - 11s 98ms/step - loss: 0.0441 - accuracy: 0.9878 - val_loss: 0.7054 - val_accuracy: 0.8760
Epoch 10/100
110/110 [==============================] - 10s 95ms/step - loss: 0.0273 - accuracy: 0.9926 - val_loss: 0.7277 - val_accuracy: 0.8620
Epoch 11/100
110/110 [==============================] - 11s 97ms/step - loss: 0.0468 - accuracy: 0.9897 - val_loss: 0.7339 - val_accuracy: 0.8660
Epoch 12/100
110/110 [==============================] - 11s 97ms/step - loss: 0.0425 - accuracy: 0.9887 - val_loss: 0.7774 - val_accuracy: 0.8720
Epoch 13/100
110/110 [==============================] - 10s 95ms/step - loss: 0.0514 - accuracy: 0.9909 - val_loss: 1.0066 - val_accuracy: 0.8700
Epoch 14/100
110/110 [==============================] - 11s 99ms/step - loss: 0.0538 - accuracy: 0.9908 - val_loss: 0.9368 - val_accuracy: 0.8720
Epoch 15/100
110/110 [==============================] - 11s 99ms/step - loss: 0.0210 - accuracy: 0.9944 - val_loss: 0.7842 - val_accuracy: 0.8680
Epoch 16/100
110/110 [==============================] - 11s 98ms/step - loss: 0.0192 - accuracy: 0.9967 - val_loss: 0.7877 - val_accuracy: 0.8760
Epoch 17/100
110/110 [==============================] - 10s 92ms/step - loss: 0.0197 - accuracy: 0.9946 - val_loss: 0.8220 - val_accuracy: 0.8680
Epoch 18/100
110/110 [==============================] - 10s 91ms/step - loss: 0.0212 - accuracy: 0.9947 - val_loss: 0.8399 - val_accuracy: 0.8420
Epoch 19/100
110/110 [==============================] - 10s 92ms/step - loss: 0.0609 - accuracy: 0.9858 - val_loss: 0.7149 - val_accuracy: 0.8680
Epoch 20/100
110/110 [==============================] - 10s 92ms/step - loss: 0.0118 - accuracy: 0.9958 - val_loss: 0.9409 - val_accuracy: 0.8700
Epoch 21/100
110/110 [==============================] - 10s 91ms/step - loss: 0.0040 - accuracy: 0.9993 - val_loss: 0.9915 - val_accuracy: 0.8680
Epoch 22/100
110/110 [==============================] - 10s 93ms/step - loss: 0.0069 - accuracy: 0.9979 - val_loss: 1.1301 - val_accuracy: 0.8700
Epoch 23/100
110/110 [==============================] - 10s 92ms/step - loss: 0.0062 - accuracy: 0.9988 - val_loss: 1.3223 - val_accuracy: 0.8480
Epoch 24/100
110/110 [==============================] - 10s 92ms/step - loss: 0.0271 - accuracy: 0.9948 - val_loss: 0.7895 - val_accuracy: 0.8720
Epoch 25/100
110/110 [==============================] - 10s 92ms/step - loss: 0.0187 - accuracy: 0.9945 - val_loss: 0.7392 - val_accuracy: 0.8580
Epoch 26/100
110/110 [==============================] - 10s 93ms/step - loss: 0.0281 - accuracy: 0.9951 - val_loss: 0.7615 - val_accuracy: 0.8560
Epoch 27/100
110/110 [==============================] - 10s 93ms/step - loss: 0.0223 - accuracy: 0.9962 - val_loss: 1.0475 - val_accuracy: 0.8540
Epoch 28/100
110/110 [==============================] - 10s 92ms/step - loss: 0.0191 - accuracy: 0.9965 - val_loss: 0.8612 - val_accuracy: 0.8700
Restoring model weights from the end of the best epoch.
Epoch 00028: early stopping
Test Accuracy: 88.20000290870667
Done!
Activation Filters Acc
0 relu 1 0.882
-------------------------------------------
Training 2: relu activation, 2 kernel size.
-------------------------------------------
Epoch 1/100
110/110 [==============================] - 15s 98ms/step - loss: 1.5191 - accuracy: 0.3592 - val_loss: 0.6674 - val_accuracy: 0.7700
Epoch 2/100
110/110 [==============================] - 10s 91ms/step - loss: 0.5972 - accuracy: 0.7956 - val_loss: 0.4412 - val_accuracy: 0.8680
Epoch 3/100
110/110 [==============================] - 10s 92ms/step - loss: 0.2754 - accuracy: 0.9134 - val_loss: 0.5438 - val_accuracy: 0.8380
Epoch 4/100
110/110 [==============================] - 10s 92ms/step - loss: 0.1619 - accuracy: 0.9497 - val_loss: 0.5266 - val_accuracy: 0.8680
Epoch 5/100
110/110 [==============================] - 10s 93ms/step - loss: 0.0962 - accuracy: 0.9700 - val_loss: 0.6383 - val_accuracy: 0.8600
Epoch 6/100
110/110 [==============================] - 10s 92ms/step - loss: 0.0612 - accuracy: 0.9833 - val_loss: 0.6902 - val_accuracy: 0.8720
Epoch 7/100
110/110 [==============================] - 10s 92ms/step - loss: 0.0782 - accuracy: 0.9827 - val_loss: 0.7112 - val_accuracy: 0.8700
Epoch 8/100
110/110 [==============================] - 10s 92ms/step - loss: 0.0704 - accuracy: 0.9818 - val_loss: 1.0214 - val_accuracy: 0.8240
Epoch 9/100
110/110 [==============================] - 10s 91ms/step - loss: 0.0919 - accuracy: 0.9786 - val_loss: 0.7743 - val_accuracy: 0.8680
Epoch 10/100
110/110 [==============================] - 10s 92ms/step - loss: 0.0310 - accuracy: 0.9898 - val_loss: 0.7885 - val_accuracy: 0.8680
Epoch 11/100
110/110 [==============================] - 10s 95ms/step - loss: 0.0260 - accuracy: 0.9937 - val_loss: 0.7305 - val_accuracy: 0.8720
Epoch 12/100
110/110 [==============================] - 10s 94ms/step - loss: 0.0245 - accuracy: 0.9911 - val_loss: 0.6378 - val_accuracy: 0.8740
Epoch 13/100
110/110 [==============================] - 10s 91ms/step - loss: 0.0322 - accuracy: 0.9911 - val_loss: 0.8051 - val_accuracy: 0.8600
Epoch 14/100
110/110 [==============================] - 10s 91ms/step - loss: 0.0332 - accuracy: 0.9937 - val_loss: 0.7549 - val_accuracy: 0.8740
Epoch 15/100
110/110 [==============================] - 10s 91ms/step - loss: 0.0200 - accuracy: 0.9952 - val_loss: 0.8510 - val_accuracy: 0.8860
Epoch 16/100
110/110 [==============================] - 10s 91ms/step - loss: 0.0257 - accuracy: 0.9944 - val_loss: 0.7560 - val_accuracy: 0.8740
Epoch 17/100
110/110 [==============================] - 10s 93ms/step - loss: 0.0212 - accuracy: 0.9955 - val_loss: 0.7273 - val_accuracy: 0.8780
Epoch 18/100
110/110 [==============================] - 10s 92ms/step - loss: 0.0152 - accuracy: 0.9946 - val_loss: 0.7476 - val_accuracy: 0.8460
Epoch 19/100
110/110 [==============================] - 10s 91ms/step - loss: 0.0236 - accuracy: 0.9918 - val_loss: 0.7515 - val_accuracy: 0.8820
Epoch 20/100
110/110 [==============================] - 10s 91ms/step - loss: 0.0149 - accuracy: 0.9969 - val_loss: 0.7394 - val_accuracy: 0.8540
Epoch 21/100
110/110 [==============================] - 10s 91ms/step - loss: 0.0282 - accuracy: 0.9911 - val_loss: 0.7298 - val_accuracy: 0.8720
Epoch 22/100
110/110 [==============================] - 10s 91ms/step - loss: 0.0125 - accuracy: 0.9960 - val_loss: 0.9161 - val_accuracy: 0.8520
Epoch 23/100
110/110 [==============================] - 10s 91ms/step - loss: 0.0186 - accuracy: 0.9967 - val_loss: 1.0669 - val_accuracy: 0.8680
Epoch 24/100
110/110 [==============================] - 10s 92ms/step - loss: 0.0058 - accuracy: 0.9983 - val_loss: 0.9762 - val_accuracy: 0.8780
Epoch 25/100
110/110 [==============================] - 10s 91ms/step - loss: 0.0047 - accuracy: 0.9986 - val_loss: 0.9517 - val_accuracy: 0.8880
Epoch 26/100
###Markdown
Summary
###Code
record.sort_values(by='Acc', ascending=False)
report = record.sort_values(by='Acc', ascending=False)
report = report.to_excel('TCN_TREC.xlsx', sheet_name='random')
###Output
_____no_output_____
###Markdown
Model 2: Word2Vec Static __Using and updating pre-trained embeddings__* In this part, we will create an Embedding layer in Tensorflow Keras using a pre-trained word embedding called Word2Vec 300-d tht has been trained 100 bilion words from Google News.* In this part, we will leave the embeddings fixed instead of updating them (dynamic). 1. __Load `Word2Vec` Pre-trained Word Embedding__
###Code
from gensim.models import KeyedVectors
word2vec = KeyedVectors.load_word2vec_format('../GoogleNews-vectors-negative300.bin', binary=True)
# Access the dense vector value for the word 'handsome'
# word2vec.word_vec('handsome') # 0.11376953
word2vec.word_vec('cool') # 1.64062500e-01
###Output
_____no_output_____
###Markdown
2. __Check number of training words present in Word2Vec__
###Code
def training_words_in_word2vector(word_to_vec_map, word_to_index):
'''
input:
word_to_vec_map: a word2vec GoogleNews-vectors-negative300.bin model loaded using gensim.models
word_to_index: word to index mapping from training set
'''
vocab_size = len(word_to_index) + 1
count = 0
# Set each row "idx" of the embedding matrix to be
# the word vector representation of the idx'th word of the vocabulary
for word, idx in word_to_index.items():
if word in word_to_vec_map:
count+=1
return print('Found {} words present from {} training vocabulary in the set of pre-trained word vector'.format(count, vocab_size))
# Separate the sentences and the labels
sentences, labels = list(corpus.sentence), list(corpus.label)
# Cleaning and Tokenization
tokenizer = Tokenizer(oov_token=oov_tok)
tokenizer.fit_on_texts(sentences)
word_index = tokenizer.word_index
training_words_in_word2vector(word2vec, word_index)
###Output
Found 7526 words present from 8761 training vocabulary in the set of pre-trained word vector
###Markdown
2. __Define a `pretrained_embedding_layer` function__
###Code
emb_mean = word2vec.vectors.mean()
emb_std = word2vec.vectors.std()
from tensorflow.keras.layers import Embedding
def pretrained_embedding_matrix(word_to_vec_map, word_to_index, emb_mean, emb_std):
'''
input:
word_to_vec_map: a word2vec GoogleNews-vectors-negative300.bin model loaded using gensim.models
word_to_index: word to index mapping from training set
'''
np.random.seed(2021)
# adding 1 to fit Keras embedding (requirement)
vocab_size = len(word_to_index) + 1
# define dimensionality of your pre-trained word vectors (= 300)
emb_dim = word_to_vec_map.word_vec('handsome').shape[0]
# initialize the matrix with generic normal distribution values
embed_matrix = np.random.normal(emb_mean, emb_std, (vocab_size, emb_dim))
# Set each row "idx" of the embedding matrix to be
# the word vector representation of the idx'th word of the vocabulary
for word, idx in word_to_index.items():
if word in word_to_vec_map:
embed_matrix[idx] = word_to_vec_map.get_vector(word)
return embed_matrix
# Test the function
w_2_i = {'<UNK>': 1, 'handsome': 2, 'cool': 3, 'shit': 4 }
em_matrix = pretrained_embedding_matrix(word2vec, w_2_i, emb_mean, emb_std)
em_matrix
###Output
_____no_output_____
###Markdown
TCN Model
###Code
def define_model_2(kernel_size = 3, activation='relu', input_dim = None,
output_dim=300, max_length = None, emb_matrix = None):
inp = Input( shape=(max_length,))
x = Embedding(input_dim=input_dim,
output_dim=output_dim,
input_length=max_length,
# Assign the embedding weight with word2vec embedding marix
weights = [emb_matrix],
# Set the weight to be not trainable (static)
trainable = False)(inp)
x = SpatialDropout1D(0.1)(x)
x = TCN(128,dilations = [1, 2, 4], return_sequences=True, activation = activation, name = 'tcn1')(x)
x = TCN(64,dilations = [1, 2, 4], return_sequences=True, activation = activation, name = 'tcn2')(x)
avg_pool = GlobalAveragePooling1D()(x)
max_pool = GlobalMaxPooling1D()(x)
conc = concatenate([avg_pool, max_pool])
conc = Dense(16, activation="relu")(conc)
conc = Dropout(0.1)(conc)
outp = Dense(6, activation="softmax")(conc)
model = Model(inputs=inp, outputs=outp)
model.compile( loss = 'sparse_categorical_crossentropy', optimizer = 'adam', metrics = ['accuracy'])
return model
model_0 = define_model_2( input_dim=1000, max_length=100, emb_matrix=np.random.rand(1000, 300))
model_0.summary()
###Output
Model: "model_13"
__________________________________________________________________________________________________
Layer (type) Output Shape Param # Connected to
==================================================================================================
input_14 (InputLayer) [(None, 100)] 0
__________________________________________________________________________________________________
embedding_13 (Embedding) (None, 100, 300) 300000 input_14[0][0]
__________________________________________________________________________________________________
spatial_dropout1d_13 (SpatialDr (None, 100, 300) 0 embedding_13[0][0]
__________________________________________________________________________________________________
tcn1 (TCN) (None, 100, 128) 279936 spatial_dropout1d_13[0][0]
__________________________________________________________________________________________________
tcn2 (TCN) (None, 100, 64) 65984 tcn1[0][0]
__________________________________________________________________________________________________
global_average_pooling1d_13 (Gl (None, 64) 0 tcn2[0][0]
__________________________________________________________________________________________________
global_max_pooling1d_13 (Global (None, 64) 0 tcn2[0][0]
__________________________________________________________________________________________________
concatenate_13 (Concatenate) (None, 128) 0 global_average_pooling1d_13[0][0]
global_max_pooling1d_13[0][0]
__________________________________________________________________________________________________
dense_26 (Dense) (None, 16) 2064 concatenate_13[0][0]
__________________________________________________________________________________________________
dropout_13 (Dropout) (None, 16) 0 dense_26[0][0]
__________________________________________________________________________________________________
dense_27 (Dense) (None, 6) 102 dropout_13[0][0]
==================================================================================================
Total params: 648,086
Trainable params: 348,086
Non-trainable params: 300,000
__________________________________________________________________________________________________
###Markdown
Train and Test the Model
###Code
# class myCallback(tf.keras.callbacks.Callback):
# # Overide the method on_epoch_end() for our benefit
# def on_epoch_end(self, epoch, logs={}):
# if (logs.get('accuracy') >= 0.9):
# print("\nReached 90% accuracy so cancelling training!")
# self.model.stop_training=True
callbacks = tf.keras.callbacks.EarlyStopping(monitor='val_accuracy', min_delta=0,
patience=20, verbose=2,
mode='auto', restore_best_weights=True)
# Parameter Initialization
trunc_type='post'
padding_type='post'
oov_tok = "<UNK>"
activations = ['relu']
filters = 100
kernel_sizes = [1, 2, 3, 4, 5, 6]
emb_mean = emb_mean
emb_std = emb_std
columns = ['Activation', 'Filters', 'Acc']
record2 = pd.DataFrame(columns = columns)
# Separate the sentences and the labels
train_x = list(corpus[corpus.split=='train'].sentence)
train_y = np.array(corpus[corpus.split=='train'].label)
test_x = list(corpus[corpus.split=='test'].sentence)
test_y = np.array(corpus[corpus.split=='test'].label)
exp = 0
for activation in activations:
for kernel_size in kernel_sizes:
exp+=1
print('-------------------------------------------')
print('Training {}: {} activation, {} kernel size.'.format(exp, activation, kernel_size))
print('-------------------------------------------')
# encode data using
# Cleaning and Tokenization
tokenizer = Tokenizer(oov_token=oov_tok)
tokenizer.fit_on_texts(train_x)
# Turn the text into sequence
training_sequences = tokenizer.texts_to_sequences(train_x)
test_sequences = tokenizer.texts_to_sequences(test_x)
max_len = max_length(training_sequences)
# Pad the sequence to have the same size
Xtrain = pad_sequences(training_sequences, maxlen=max_len, padding=padding_type, truncating=trunc_type)
Xtest = pad_sequences(test_sequences, maxlen=max_len, padding=padding_type, truncating=trunc_type)
word_index = tokenizer.word_index
vocab_size = len(word_index)+1
emb_matrix = pretrained_embedding_matrix(word2vec, word_index, emb_mean, emb_std)
# Define the input shape
model = define_model_2(kernel_size, activation, input_dim=vocab_size,
max_length=max_len, emb_matrix=emb_matrix)
# Train the model and initialize test accuracy with 0
acc = 0
while(acc<0.6):
model.fit(Xtrain, train_y, batch_size=50, epochs=100, verbose=1,
callbacks=[callbacks], validation_data=(Xtest, test_y))
# evaluate the model
loss, acc = model.evaluate(Xtest, test_y, verbose=0)
print('Test Accuracy: {}'.format(acc*100))
if (acc<0.6):
print('The model suffered from local minimum. Retrain the model!')
model = define_model_2(kernel_size, activation, input_dim=vocab_size,
max_length=max_len, emb_matrix=emb_matrix)
else:
print('Done!')
parameters = [activation, kernel_size]
entries = parameters + [acc]
temp = pd.DataFrame([entries], columns=columns)
record2 = record2.append(temp, ignore_index=True)
print()
print(record2)
print()
###Output
-------------------------------------------
Training 1: relu activation, 1 kernel size.
-------------------------------------------
Epoch 1/100
110/110 [==============================] - 8s 51ms/step - loss: 1.6586 - accuracy: 0.2539 - val_loss: 1.1601 - val_accuracy: 0.5320
Epoch 2/100
110/110 [==============================] - 7s 61ms/step - loss: 1.0465 - accuracy: 0.5740 - val_loss: 0.7761 - val_accuracy: 0.7300
Epoch 3/100
110/110 [==============================] - 7s 63ms/step - loss: 0.7100 - accuracy: 0.7412 - val_loss: 0.6517 - val_accuracy: 0.8280
Epoch 4/100
110/110 [==============================] - 7s 63ms/step - loss: 0.5298 - accuracy: 0.8058 - val_loss: 0.4062 - val_accuracy: 0.8560
Epoch 5/100
110/110 [==============================] - 6s 58ms/step - loss: 0.3496 - accuracy: 0.8829 - val_loss: 0.3442 - val_accuracy: 0.8820
Epoch 6/100
110/110 [==============================] - 6s 57ms/step - loss: 0.2555 - accuracy: 0.9135 - val_loss: 0.4897 - val_accuracy: 0.8440
Epoch 7/100
110/110 [==============================] - 6s 58ms/step - loss: 0.3203 - accuracy: 0.8888 - val_loss: 0.3376 - val_accuracy: 0.8940
Epoch 8/100
110/110 [==============================] - 6s 58ms/step - loss: 0.1902 - accuracy: 0.9335 - val_loss: 0.3990 - val_accuracy: 0.8920
Epoch 9/100
110/110 [==============================] - 6s 58ms/step - loss: 0.1398 - accuracy: 0.9542 - val_loss: 0.3339 - val_accuracy: 0.9100
Epoch 10/100
110/110 [==============================] - 6s 59ms/step - loss: 0.1282 - accuracy: 0.9558 - val_loss: 0.4657 - val_accuracy: 0.8580
Epoch 11/100
110/110 [==============================] - 6s 58ms/step - loss: 0.1166 - accuracy: 0.9627 - val_loss: 0.3652 - val_accuracy: 0.8960
Epoch 12/100
110/110 [==============================] - 6s 58ms/step - loss: 0.1231 - accuracy: 0.9570 - val_loss: 0.4489 - val_accuracy: 0.8860
Epoch 13/100
110/110 [==============================] - 6s 57ms/step - loss: 0.1003 - accuracy: 0.9682 - val_loss: 0.4257 - val_accuracy: 0.9040
Epoch 14/100
110/110 [==============================] - 7s 62ms/step - loss: 0.0820 - accuracy: 0.9761 - val_loss: 0.5239 - val_accuracy: 0.8820
Epoch 15/100
110/110 [==============================] - 6s 59ms/step - loss: 0.0968 - accuracy: 0.9671 - val_loss: 0.4569 - val_accuracy: 0.8940
Epoch 16/100
110/110 [==============================] - 7s 62ms/step - loss: 0.0669 - accuracy: 0.9785 - val_loss: 0.4383 - val_accuracy: 0.9080
Epoch 17/100
110/110 [==============================] - 7s 63ms/step - loss: 0.0636 - accuracy: 0.9811 - val_loss: 0.4992 - val_accuracy: 0.7960
Epoch 18/100
110/110 [==============================] - 7s 64ms/step - loss: 0.1021 - accuracy: 0.9739 - val_loss: 0.4608 - val_accuracy: 0.8840
Epoch 19/100
110/110 [==============================] - 6s 57ms/step - loss: 0.0652 - accuracy: 0.9793 - val_loss: 0.5732 - val_accuracy: 0.8940
Epoch 20/100
110/110 [==============================] - 6s 57ms/step - loss: 0.0411 - accuracy: 0.9854 - val_loss: 0.5148 - val_accuracy: 0.9020
Epoch 21/100
110/110 [==============================] - 6s 58ms/step - loss: 0.0363 - accuracy: 0.9884 - val_loss: 0.5309 - val_accuracy: 0.9060
Epoch 22/100
110/110 [==============================] - 7s 59ms/step - loss: 0.0396 - accuracy: 0.9859 - val_loss: 0.5638 - val_accuracy: 0.8940
Epoch 23/100
110/110 [==============================] - 6s 58ms/step - loss: 0.0473 - accuracy: 0.9867 - val_loss: 0.4729 - val_accuracy: 0.9180
Epoch 24/100
110/110 [==============================] - 6s 58ms/step - loss: 0.0314 - accuracy: 0.9901 - val_loss: 0.5098 - val_accuracy: 0.8980
Epoch 25/100
110/110 [==============================] - 6s 57ms/step - loss: 0.0501 - accuracy: 0.9860 - val_loss: 0.5787 - val_accuracy: 0.8840
Epoch 26/100
110/110 [==============================] - 6s 57ms/step - loss: 0.0603 - accuracy: 0.9840 - val_loss: 0.5067 - val_accuracy: 0.9120
Epoch 27/100
110/110 [==============================] - 6s 59ms/step - loss: 0.0418 - accuracy: 0.9849 - val_loss: 0.4713 - val_accuracy: 0.9340
Epoch 28/100
110/110 [==============================] - 7s 60ms/step - loss: 0.0552 - accuracy: 0.9842 - val_loss: 0.3812 - val_accuracy: 0.9320
Epoch 29/100
110/110 [==============================] - 6s 59ms/step - loss: 0.0341 - accuracy: 0.9904 - val_loss: 0.4757 - val_accuracy: 0.9100
Epoch 30/100
110/110 [==============================] - 7s 59ms/step - loss: 0.0362 - accuracy: 0.9899 - val_loss: 0.5092 - val_accuracy: 0.9160
Epoch 31/100
110/110 [==============================] - 6s 58ms/step - loss: 0.0276 - accuracy: 0.9931 - val_loss: 0.5957 - val_accuracy: 0.9240
Epoch 32/100
110/110 [==============================] - 7s 60ms/step - loss: 0.0410 - accuracy: 0.9916 - val_loss: 0.3946 - val_accuracy: 0.9140
Epoch 33/100
110/110 [==============================] - 7s 60ms/step - loss: 0.0313 - accuracy: 0.9930 - val_loss: 0.3733 - val_accuracy: 0.9220
Epoch 34/100
110/110 [==============================] - 7s 60ms/step - loss: 0.0349 - accuracy: 0.9910 - val_loss: 0.4861 - val_accuracy: 0.9320
Epoch 35/100
110/110 [==============================] - 7s 59ms/step - loss: 0.0194 - accuracy: 0.9937 - val_loss: 0.5066 - val_accuracy: 0.9180
Epoch 36/100
110/110 [==============================] - 7s 60ms/step - loss: 0.0232 - accuracy: 0.9950 - val_loss: 0.5587 - val_accuracy: 0.9100
Epoch 37/100
110/110 [==============================] - 6s 58ms/step - loss: 0.0307 - accuracy: 0.9929 - val_loss: 0.6107 - val_accuracy: 0.9100
Epoch 38/100
110/110 [==============================] - 6s 57ms/step - loss: 0.0304 - accuracy: 0.9912 - val_loss: 0.9091 - val_accuracy: 0.8220
Epoch 39/100
110/110 [==============================] - 6s 59ms/step - loss: 0.0457 - accuracy: 0.9850 - val_loss: 0.5512 - val_accuracy: 0.8380
Epoch 40/100
110/110 [==============================] - 7s 61ms/step - loss: 0.0442 - accuracy: 0.9895 - val_loss: 0.4444 - val_accuracy: 0.9180
Epoch 41/100
110/110 [==============================] - 7s 66ms/step - loss: 0.0360 - accuracy: 0.9906 - val_loss: 0.4632 - val_accuracy: 0.9360
Epoch 42/100
110/110 [==============================] - 7s 62ms/step - loss: 0.0410 - accuracy: 0.9892 - val_loss: 0.6614 - val_accuracy: 0.9160
Epoch 43/100
110/110 [==============================] - 7s 60ms/step - loss: 0.0246 - accuracy: 0.9927 - val_loss: 0.8239 - val_accuracy: 0.7980
Epoch 44/100
110/110 [==============================] - 7s 60ms/step - loss: 0.0250 - accuracy: 0.9928 - val_loss: 0.6767 - val_accuracy: 0.9140
Epoch 45/100
110/110 [==============================] - 7s 60ms/step - loss: 0.0129 - accuracy: 0.9965 - val_loss: 0.7094 - val_accuracy: 0.9120
Epoch 46/100
110/110 [==============================] - 7s 60ms/step - loss: 0.0242 - accuracy: 0.9937 - val_loss: 0.5325 - val_accuracy: 0.8900
Epoch 47/100
110/110 [==============================] - 7s 60ms/step - loss: 0.0280 - accuracy: 0.9913 - val_loss: 0.5926 - val_accuracy: 0.8980
Epoch 48/100
110/110 [==============================] - 7s 60ms/step - loss: 0.0230 - accuracy: 0.9925 - val_loss: 0.6531 - val_accuracy: 0.9060
Epoch 49/100
110/110 [==============================] - 7s 61ms/step - loss: 0.0185 - accuracy: 0.9940 - val_loss: 0.5960 - val_accuracy: 0.9060
Epoch 50/100
110/110 [==============================] - 7s 62ms/step - loss: 0.0443 - accuracy: 0.9864 - val_loss: 0.6159 - val_accuracy: 0.9220
Epoch 51/100
110/110 [==============================] - 7s 59ms/step - loss: 0.0210 - accuracy: 0.9936 - val_loss: 0.5719 - val_accuracy: 0.8980
Epoch 52/100
110/110 [==============================] - 6s 58ms/step - loss: 0.1073 - accuracy: 0.9719 - val_loss: 0.4511 - val_accuracy: 0.8800
Epoch 53/100
110/110 [==============================] - 6s 58ms/step - loss: 0.0467 - accuracy: 0.9906 - val_loss: 0.4375 - val_accuracy: 0.9020
Epoch 54/100
110/110 [==============================] - 7s 60ms/step - loss: 0.0325 - accuracy: 0.9927 - val_loss: 0.5963 - val_accuracy: 0.9040
Epoch 55/100
110/110 [==============================] - 7s 68ms/step - loss: 0.0148 - accuracy: 0.9966 - val_loss: 0.6056 - val_accuracy: 0.9080
Epoch 56/100
###Markdown
Summary
###Code
record2.sort_values(by='Acc', ascending=False)
report = record2.sort_values(by='Acc', ascending=False)
report = report.to_excel('TCN_TREC_2.xlsx', sheet_name='static')
###Output
_____no_output_____
###Markdown
Model 3: Word2Vec - Dynamic * In this part, we will fine tune the embeddings while training (dynamic). TCN Model
###Code
def define_model_3(kernel_size = 3, activation='relu', input_dim = None,
output_dim=300, max_length = None, emb_matrix = None):
inp = Input( shape=(max_length,))
x = Embedding(input_dim=input_dim,
output_dim=output_dim,
input_length=max_length,
# Assign the embedding weight with word2vec embedding marix
weights = [emb_matrix],
# Set the weight to be not trainable (static)
trainable = True)(inp)
x = SpatialDropout1D(0.1)(x)
x = TCN(128,dilations = [1, 2, 4], return_sequences=True, activation = activation, name = 'tcn1')(x)
x = TCN(64,dilations = [1, 2, 4], return_sequences=True, activation = activation, name = 'tcn2')(x)
avg_pool = GlobalAveragePooling1D()(x)
max_pool = GlobalMaxPooling1D()(x)
conc = concatenate([avg_pool, max_pool])
conc = Dense(16, activation="relu")(conc)
conc = Dropout(0.1)(conc)
outp = Dense(6, activation="softmax")(conc)
model = Model(inputs=inp, outputs=outp)
model.compile( loss = 'sparse_categorical_crossentropy', optimizer = 'adam', metrics = ['accuracy'])
return model
model_0 = define_model_3( input_dim=1000, max_length=100, emb_matrix=np.random.rand(1000, 300))
model_0.summary()
###Output
Model: "model_20"
__________________________________________________________________________________________________
Layer (type) Output Shape Param # Connected to
==================================================================================================
input_21 (InputLayer) [(None, 100)] 0
__________________________________________________________________________________________________
embedding_20 (Embedding) (None, 100, 300) 300000 input_21[0][0]
__________________________________________________________________________________________________
spatial_dropout1d_20 (SpatialDr (None, 100, 300) 0 embedding_20[0][0]
__________________________________________________________________________________________________
tcn1 (TCN) (None, 100, 128) 279936 spatial_dropout1d_20[0][0]
__________________________________________________________________________________________________
tcn2 (TCN) (None, 100, 64) 65984 tcn1[0][0]
__________________________________________________________________________________________________
global_average_pooling1d_20 (Gl (None, 64) 0 tcn2[0][0]
__________________________________________________________________________________________________
global_max_pooling1d_20 (Global (None, 64) 0 tcn2[0][0]
__________________________________________________________________________________________________
concatenate_20 (Concatenate) (None, 128) 0 global_average_pooling1d_20[0][0]
global_max_pooling1d_20[0][0]
__________________________________________________________________________________________________
dense_40 (Dense) (None, 16) 2064 concatenate_20[0][0]
__________________________________________________________________________________________________
dropout_20 (Dropout) (None, 16) 0 dense_40[0][0]
__________________________________________________________________________________________________
dense_41 (Dense) (None, 6) 102 dropout_20[0][0]
==================================================================================================
Total params: 648,086
Trainable params: 648,086
Non-trainable params: 0
__________________________________________________________________________________________________
###Markdown
Train and Test the Model
###Code
class myCallback(tf.keras.callbacks.Callback):
# Overide the method on_epoch_end() for our benefit
def on_epoch_end(self, epoch, logs={}):
if (logs.get('accuracy') > 0.93):
print("\nReached 93% accuracy so cancelling training!")
self.model.stop_training=True
callbacks = tf.keras.callbacks.EarlyStopping(monitor='val_accuracy', min_delta=0,
patience=20, verbose=2,
mode='auto', restore_best_weights=True)
# Parameter Initialization
trunc_type='post'
padding_type='post'
oov_tok = "<UNK>"
activations = ['relu']
filters = 100
kernel_sizes = [1, 2, 3, 4, 5, 6]
emb_mean = emb_mean
emb_std = emb_std
columns = ['Activation', 'Filters', 'Acc']
record3 = pd.DataFrame(columns = columns)
# Separate the sentences and the labels
train_x = list(corpus[corpus.split=='train'].sentence)
train_y = np.array(corpus[corpus.split=='train'].label)
test_x = list(corpus[corpus.split=='test'].sentence)
test_y = np.array(corpus[corpus.split=='test'].label)
exp = 0
for activation in activations:
for kernel_size in kernel_sizes:
exp+=1
print('-------------------------------------------')
print('Training {}: {} activation, {} kernel size.'.format(exp, activation, kernel_size))
print('-------------------------------------------')
# encode data using
# Cleaning and Tokenization
tokenizer = Tokenizer(oov_token=oov_tok)
tokenizer.fit_on_texts(train_x)
# Turn the text into sequence
training_sequences = tokenizer.texts_to_sequences(train_x)
test_sequences = tokenizer.texts_to_sequences(test_x)
max_len = max_length(training_sequences)
# Pad the sequence to have the same size
Xtrain = pad_sequences(training_sequences, maxlen=max_len, padding=padding_type, truncating=trunc_type)
Xtest = pad_sequences(test_sequences, maxlen=max_len, padding=padding_type, truncating=trunc_type)
word_index = tokenizer.word_index
vocab_size = len(word_index)+1
emb_matrix = pretrained_embedding_matrix(word2vec, word_index, emb_mean, emb_std)
# Define the input shape
model = define_model_3(kernel_size, activation, input_dim=vocab_size,
max_length=max_len, emb_matrix=emb_matrix)
# Train the model and initialize test accuracy with 0
acc = 0
while(acc<0.6):
model.fit(Xtrain, train_y, batch_size=50, epochs=200, verbose=1,
callbacks=[callbacks], validation_data=(Xtest, test_y))
# evaluate the model
loss, acc = model.evaluate(Xtest, test_y, verbose=0)
print('Test Accuracy: {}'.format(acc*100))
if (acc<0.6):
print('The model suffered from local minimum. Retrain the model!')
model = define_model_3(kernel_size, activation, input_dim=vocab_size,
max_length=max_len, emb_matrix=emb_matrix)
else:
print('Done!')
parameters = [activation, kernel_size]
entries = parameters + [acc]
temp = pd.DataFrame([entries], columns=columns)
record3 = record3.append(temp, ignore_index=True)
print()
print(record3)
print()
###Output
-------------------------------------------
Training 1: relu activation, 1 kernel size.
-------------------------------------------
Epoch 1/200
110/110 [==============================] - 16s 105ms/step - loss: 1.7516 - accuracy: 0.2901 - val_loss: 0.9225 - val_accuracy: 0.6700
Epoch 2/200
110/110 [==============================] - 11s 104ms/step - loss: 0.8424 - accuracy: 0.6706 - val_loss: 0.6787 - val_accuracy: 0.7800
Epoch 3/200
110/110 [==============================] - 11s 100ms/step - loss: 0.4505 - accuracy: 0.8515 - val_loss: 0.3809 - val_accuracy: 0.8900
Epoch 4/200
110/110 [==============================] - 12s 109ms/step - loss: 0.2638 - accuracy: 0.9109 - val_loss: 0.3444 - val_accuracy: 0.8740
Epoch 5/200
110/110 [==============================] - 11s 104ms/step - loss: 0.1397 - accuracy: 0.9541 - val_loss: 0.6288 - val_accuracy: 0.8160
Epoch 6/200
110/110 [==============================] - 10s 95ms/step - loss: 0.1158 - accuracy: 0.9593 - val_loss: 0.4092 - val_accuracy: 0.9000
Epoch 7/200
110/110 [==============================] - 11s 96ms/step - loss: 0.0834 - accuracy: 0.9692 - val_loss: 0.4955 - val_accuracy: 0.9080
Epoch 8/200
110/110 [==============================] - 10s 95ms/step - loss: 0.0843 - accuracy: 0.9701 - val_loss: 1.0062 - val_accuracy: 0.8400
Epoch 9/200
110/110 [==============================] - 10s 95ms/step - loss: 0.0688 - accuracy: 0.9756 - val_loss: 0.6516 - val_accuracy: 0.8860
Epoch 10/200
110/110 [==============================] - 10s 94ms/step - loss: 0.0329 - accuracy: 0.9890 - val_loss: 0.7158 - val_accuracy: 0.8780
Epoch 11/200
110/110 [==============================] - 10s 95ms/step - loss: 0.0713 - accuracy: 0.9793 - val_loss: 0.7521 - val_accuracy: 0.8560
Epoch 12/200
110/110 [==============================] - 10s 94ms/step - loss: 0.0685 - accuracy: 0.9775 - val_loss: 0.5951 - val_accuracy: 0.8860
Epoch 13/200
110/110 [==============================] - 10s 94ms/step - loss: 0.0335 - accuracy: 0.9900 - val_loss: 0.8378 - val_accuracy: 0.8580
Epoch 14/200
110/110 [==============================] - 10s 95ms/step - loss: 0.0353 - accuracy: 0.9885 - val_loss: 0.6158 - val_accuracy: 0.8900
Epoch 15/200
110/110 [==============================] - 10s 95ms/step - loss: 0.0195 - accuracy: 0.9926 - val_loss: 0.7227 - val_accuracy: 0.8780
Epoch 16/200
110/110 [==============================] - 10s 94ms/step - loss: 0.0596 - accuracy: 0.9841 - val_loss: 1.0022 - val_accuracy: 0.8820
Epoch 17/200
110/110 [==============================] - 10s 94ms/step - loss: 0.0531 - accuracy: 0.9858 - val_loss: 0.9123 - val_accuracy: 0.8540
Epoch 18/200
110/110 [==============================] - 10s 94ms/step - loss: 0.0464 - accuracy: 0.9856 - val_loss: 0.6033 - val_accuracy: 0.9020
Epoch 19/200
110/110 [==============================] - 11s 100ms/step - loss: 0.0170 - accuracy: 0.9955 - val_loss: 0.8228 - val_accuracy: 0.8700
Epoch 20/200
110/110 [==============================] - 12s 108ms/step - loss: 0.3418 - accuracy: 0.9169 - val_loss: 0.5869 - val_accuracy: 0.8860
Epoch 21/200
110/110 [==============================] - 11s 98ms/step - loss: 0.0323 - accuracy: 0.9936 - val_loss: 0.7167 - val_accuracy: 0.8600
Epoch 22/200
110/110 [==============================] - 12s 105ms/step - loss: 0.0243 - accuracy: 0.9953 - val_loss: 1.2085 - val_accuracy: 0.8400
Epoch 23/200
110/110 [==============================] - 11s 104ms/step - loss: 0.0277 - accuracy: 0.9929 - val_loss: 0.9070 - val_accuracy: 0.8740
Epoch 24/200
110/110 [==============================] - 11s 100ms/step - loss: 0.0178 - accuracy: 0.9947 - val_loss: 0.9643 - val_accuracy: 0.8660
Epoch 25/200
110/110 [==============================] - 11s 101ms/step - loss: 0.0189 - accuracy: 0.9969 - val_loss: 0.8347 - val_accuracy: 0.8840
Epoch 26/200
110/110 [==============================] - 11s 97ms/step - loss: 0.0285 - accuracy: 0.9942 - val_loss: 0.9499 - val_accuracy: 0.8700
Epoch 27/200
110/110 [==============================] - 12s 111ms/step - loss: 0.0492 - accuracy: 0.9936 - val_loss: 0.8579 - val_accuracy: 0.8820
Restoring model weights from the end of the best epoch.
Epoch 00027: early stopping
Test Accuracy: 90.79999923706055
Done!
Activation Filters Acc
0 relu 1 0.908
-------------------------------------------
Training 2: relu activation, 2 kernel size.
-------------------------------------------
Epoch 1/200
110/110 [==============================] - 17s 105ms/step - loss: 1.9122 - accuracy: 0.2446 - val_loss: 1.0028 - val_accuracy: 0.6640
Epoch 2/200
110/110 [==============================] - 11s 96ms/step - loss: 0.9632 - accuracy: 0.6106 - val_loss: 0.4967 - val_accuracy: 0.8380
Epoch 3/200
110/110 [==============================] - 11s 101ms/step - loss: 0.4720 - accuracy: 0.8409 - val_loss: 0.3873 - val_accuracy: 0.8980
Epoch 4/200
110/110 [==============================] - 10s 95ms/step - loss: 0.2550 - accuracy: 0.9234 - val_loss: 0.4167 - val_accuracy: 0.8920
Epoch 5/200
110/110 [==============================] - 10s 94ms/step - loss: 0.1632 - accuracy: 0.9436 - val_loss: 0.4496 - val_accuracy: 0.8940
Epoch 6/200
110/110 [==============================] - 10s 94ms/step - loss: 0.1305 - accuracy: 0.9593 - val_loss: 0.6310 - val_accuracy: 0.8720
Epoch 7/200
110/110 [==============================] - 10s 93ms/step - loss: 0.0792 - accuracy: 0.9763 - val_loss: 0.5516 - val_accuracy: 0.8900
Epoch 8/200
110/110 [==============================] - 10s 93ms/step - loss: 0.0576 - accuracy: 0.9802 - val_loss: 0.5802 - val_accuracy: 0.8920
Epoch 9/200
110/110 [==============================] - 10s 94ms/step - loss: 0.0583 - accuracy: 0.9813 - val_loss: 0.7471 - val_accuracy: 0.8820
Epoch 10/200
110/110 [==============================] - 10s 93ms/step - loss: 0.1333 - accuracy: 0.9656 - val_loss: 0.8602 - val_accuracy: 0.8580
Epoch 11/200
110/110 [==============================] - 10s 93ms/step - loss: 0.0518 - accuracy: 0.9847 - val_loss: 0.4637 - val_accuracy: 0.9000
Epoch 12/200
110/110 [==============================] - 10s 94ms/step - loss: 0.0357 - accuracy: 0.9863 - val_loss: 0.7575 - val_accuracy: 0.8780
Epoch 13/200
110/110 [==============================] - 10s 94ms/step - loss: 0.0510 - accuracy: 0.9859 - val_loss: 0.5935 - val_accuracy: 0.9060
Epoch 14/200
110/110 [==============================] - 10s 94ms/step - loss: 0.0303 - accuracy: 0.9910 - val_loss: 0.6504 - val_accuracy: 0.9020
Epoch 15/200
110/110 [==============================] - 10s 94ms/step - loss: 0.0282 - accuracy: 0.9883 - val_loss: 0.9312 - val_accuracy: 0.8860
Epoch 16/200
110/110 [==============================] - 10s 93ms/step - loss: 0.0662 - accuracy: 0.9835 - val_loss: 0.6040 - val_accuracy: 0.8900
Epoch 17/200
110/110 [==============================] - 10s 94ms/step - loss: 0.0422 - accuracy: 0.9873 - val_loss: 0.6847 - val_accuracy: 0.8920
Epoch 18/200
110/110 [==============================] - 10s 95ms/step - loss: 0.0343 - accuracy: 0.9886 - val_loss: 0.9597 - val_accuracy: 0.8820
Epoch 19/200
110/110 [==============================] - 10s 96ms/step - loss: 0.0143 - accuracy: 0.9947 - val_loss: 1.1239 - val_accuracy: 0.8820
Epoch 20/200
110/110 [==============================] - 10s 95ms/step - loss: 0.0251 - accuracy: 0.9929 - val_loss: 1.1022 - val_accuracy: 0.8700
Epoch 21/200
110/110 [==============================] - 10s 94ms/step - loss: 0.0283 - accuracy: 0.9929 - val_loss: 0.9089 - val_accuracy: 0.8860
Epoch 22/200
110/110 [==============================] - 10s 94ms/step - loss: 0.0480 - accuracy: 0.9901 - val_loss: 1.0349 - val_accuracy: 0.8080
Epoch 23/200
110/110 [==============================] - 10s 93ms/step - loss: 0.0245 - accuracy: 0.9933 - val_loss: 1.0862 - val_accuracy: 0.8780
Epoch 24/200
110/110 [==============================] - 10s 94ms/step - loss: 0.0177 - accuracy: 0.9935 - val_loss: 1.0507 - val_accuracy: 0.7780
Epoch 25/200
110/110 [==============================] - 10s 94ms/step - loss: 0.0175 - accuracy: 0.9971 - val_loss: 0.8097 - val_accuracy: 0.8760
Epoch 26/200
110/110 [==============================] - 10s 94ms/step - loss: 0.0214 - accuracy: 0.9939 - val_loss: 0.7458 - val_accuracy: 0.8840
Epoch 27/200
###Markdown
Summary
###Code
record3.sort_values(by='Acc', ascending=False)
report = record3.sort_values(by='Acc', ascending=False)
report = report.to_excel('TCN_TREC_3.xlsx', sheet_name='dynamic')
###Output
_____no_output_____ |
gs_quant/documentation/02_pricing_and_risk/01_scenarios_and_contexts/examples/02_market_shock/010205_vega_shock_equivalent.ipynb | ###Markdown
Risk measures are short-hand for a set of predefined scenarios. Let's look at how at to get the `IRVegaParallel` value using a `MarketDataShockBasedScenario`. We calculate delta as a 2-sided 1bp bump.
###Code
swaption = IRSwaption(PayReceive.Pay, '10y', Currency.USD, notional_amount=10e6)
swaption.resolve()
r_shock_bp = 1/10000
ir_scenario_up = MarketDataShockBasedScenario({MarketDataPattern('IR Vol'):
MarketDataShock(MarketDataShockType.Absolute, r_shock_bp)})
ir_scenario_down = MarketDataShockBasedScenario({MarketDataPattern('IR Vol'):
MarketDataShock(MarketDataShockType.Absolute, -r_shock_bp)})
with PricingContext():
vega = swaption.calc(IRVegaParallel)
with ir_scenario_up:
up = swaption.dollar_price()
with ir_scenario_down:
down = swaption.dollar_price()
combined = (up.result() - down.result())/2
# should give the same value
combined - delta.result()
print(f'Vega direct={vega.result():.0f}, Vega by shocks={((up.result() - down.result())/2):.0f}')
###Output
_____no_output_____ |
Code/Notebooks/[email protected] | ###Markdown
- computing of transparent decisions (XAI), with the help of Deep Learning methodsThe following Notebooks represents the Prototype which is introduced in the Bachelorthises "Automatic image recognition in upload filters - computing of transparent decisions (XAI), with the help of Deep Learning methods". The Prototype Implementation was created to show how the SHAP library can be used to make convolutional networks decisions transparent.Therefore it uses a VGG16 architecture and transfer learning as can be seen in the corresponding thesis. To save hardware resources, this Notebook is optimized for Google collab. An interactive online ide. With the help of Jupyter Notebooks, it allows using of google hardware resources to work and machine learning tasks.
###Code
from keras.applications.vgg16 import VGG16
from keras.applications.vgg16 import preprocess_input
import keras.backend as K
import numpy as np
import json
import shap
import os
import shutil
from keras.preprocessing.image import ImageDataGenerator
#print(keras.__version__)
#print(shap.__version__)
# Test of the SHAP Library
## Use the example case from the github page
###Output
_____no_output_____ |
action_gap_rl/notebooks/pendulum_collect_data.ipynb | ###Markdown
***Copyright 2020 Google LLC.***Licensed under the Apache License, Version 2.0 (the "License");
###Code
#@title Default title text
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
import gym
import tensorflow.compat.v2 as tf
import numpy as np
import pickle
import imp
import getpass
import os
import random
import string
from action_gap_rl import replay
from action_gap_rl import value as value_lib
from action_gap_rl.policies import layers_lib
replay = imp.reload(replay)
value_lib = imp.reload(value_lib)
layers_lib = imp.reload(layers_lib)
tf.enable_v2_behavior()
class AttrDict(dict):
def __init__(self, *args, **kwargs):
super(AttrDict, self).__init__(*args, **kwargs)
self.__dict__ = self
def to_dict(d):
if isinstance(d, AttrDict):
return {k: to_dict(v) for k, v in d.items()}
return d
def filter_bool(lst, mask):
return [lst[i] for i in range(len(lst)) if mask[i]]
def rand_str(N):
return ''.join(random.choice(string.ascii_uppercase + string.digits) for _ in range(N))
class BehaviorPolicy(tf.keras.Model):
"""A policy that takes an arbitrary function as the un-normalized log pdf."""
def __init__(self, config, name=None):
super(BehaviorPolicy, self).__init__(
name=name or self.__class__.__name__)
self._config = config
self.num_actions = config.num_actions
if 'initializer' in config:
init = config.initializer
else:
init = tf.keras.initializers.glorot_uniform()
hidden_widths = config.hidden_widths
if config.embed:
transformation_layers = [layers_lib.soft_hot_layer(**config.embed)]
else:
transformation_layers = []
self._body = tf.keras.Sequential(
transformation_layers
+ [tf.keras.layers.Dense(w, activation='relu', kernel_initializer=init) for w in hidden_widths]
+ [tf.keras.layers.Dense(self.num_actions, activation=None, kernel_initializer=init)]
)
def call(self, states):
return tf.argmax(self._body(tf.expand_dims(states, axis=0)), axis=-1).numpy()[0]
class ActionAdaptor(object):
def __init__(self, env, actions={0:-2., 1:2.}, t_res=1):
self.env = env
self.actions = actions
self.t_res = t_res
assert t_res >= 1
def step(self, a):
for _ in range(self.t_res):
result = self.env.step([self.actions[a]])
return result
def reset(self):
return self.env.reset()
@property
def unwrapped(self):
return self.env.unwrapped
@property
def action_space(self):
return gym.spaces.Discrete(2)
import copy
def policy_returns_with_horizon(env, state, policy, horizon, irresolution=1, forced_actions=()):
env = copy.deepcopy(env)
R = 0.
for t in range(horizon):
if t < len(forced_actions):
a = forced_actions[t]
else:
a = policy(state)
for _ in range(irresolution):
state, reward, term, _ = env.step(a)
R += reward
if term: break
return R
# TODO: Horizon H returns under optimal and behavior policies.
# TODO: more efficient episode sampling using an ensemble of behavior policies
WRITE_OUT = True #@param
FILTER = True #@param
compute_behavior_policy_returns = True #@param
## compute_optimal_policy_returns = False #@param
num_episodes = 30 #@param
num_datasets = 2 #@param
episode_length = 200 #@param
temporal_resolution = 10 #@param
horizons = [1, 5, 10] #@param
# file_name = "v3/pendulum_a2_t10_nnp_eval" #@param
file_name = "v3/pendulum_test" #@param
RENDER = False #@param
env = ActionAdaptor(gym.make('Pendulum-v0'))
embed=layers_lib.obs_embedding_kwargs(
20,
bounds=((-1,1),(-1,1),(0,2*np.pi)),
variance=[1.]*3,
spillover=0.05,
)
# embed=None
data_keys = []
if compute_behavior_policy_returns:
for h in horizons:
data_keys.extend(['pi0_h={}/R0'.format(h), 'pi0_h={}/R1'.format(h)])
memory = replay.Memory(data_keys)
for dataset_index in range(num_datasets):
print('dataset index =', dataset_index)
for _ in range(num_episodes):
behavior_policy = BehaviorPolicy(AttrDict(
num_actions=2,
initializer=tf.keras.initializers.glorot_normal(),
embed=embed,
hidden_widths=[64]),
name='policy_'+rand_str(10))
# collect a trajectory
obs = env.reset()
memory.log_init(obs)
for _ in range(episode_length // temporal_resolution):
if RENDER: env.render()
act = behavior_policy(obs)
for rep in range(temporal_resolution):
if compute_behavior_policy_returns:
data = {}
for h in horizons:
if rep % h == 0:
r0, r1 = [
policy_returns_with_horizon(
env,
obs,
behavior_policy,
horizon=horizon,
irresolution=temporal_resolution,
forced_actions=(a,))
for a in (0, 1)]
data.update({'pi0_h={}/R0'.format(h): r0, 'pi0_h={}/R1'.format(h): r1})
else:
data.update({'pi0_h={}/R0'.format(h): 0., 'pi0_h={}/R1'.format(h): 0.})
else:
data = {}
next_obs, reward, term, _ = env.step(act)
memory.log_experience(obs, act, reward, next_obs, data=data)
obs = next_obs
if term:
break
if RENDER: env.render()
print('done simulating')
if FILTER:
ma = np.mean(memory.actions, axis=1)
mask = np.logical_and(ma>=0.33, ma<=.66)
print('Num episodes retained:', np.count_nonzero(mask))
print('Returns:', np.sum(memory.rewards, axis=1)[mask].tolist())
memory.observations = filter_bool(memory.observations, mask)
memory.actions = filter_bool(memory.actions, mask)
memory.rewards = filter_bool(memory.rewards, mask)
print('done filtering')
if WRITE_OUT:
s = memory.serialize()
# Make directory.
user = getpass.getuser()
path = '/tmp/action_gap_rl/datasets'.format(user)
os.makedirs(path)
# Save pickle file
with open(
os.path.join(path, '{}.{}.pickle'.format(file_name, dataset_index)),
'wb') as f:
f.write(s)
# Sanity check serialization.
m2 = replay.Memory()
m2.unserialize(s)
print(np.array_equal(m2.entered_states(), memory.entered_states()))
print(np.array_equal(m2.exited_states(), memory.exited_states()))
print(np.array_equal(m2.attempted_actions(), memory.attempted_actions()))
print(np.array_equal(m2.observed_rewards(), memory.observed_rewards()))
print('\n\n')
###Output
_____no_output_____ |
dmu1/dmu1_ml_SGP/1.2_KIDS.ipynb | ###Markdown
HATLAS-SGP master catalogue Preparation of KIDS/VST dataKilo Degree Survey/VLT Survey Telescope catalogue: the catalogue comes from `dmu0_KIDS`.In the catalogue, we keep:- The identifier (it's unique in the catalogue);- The position;- The stellarity;- The aperture corrected aperture magnitude in each band (10 pixels = 2")- The Petrosian magnitude to be used as total magnitude (no “auto” magnitude is provided).We take 2014 as the observation year from a typical image header.
###Code
from herschelhelp_internal import git_version
print("This notebook was run with herschelhelp_internal version: \n{}".format(git_version()))
import datetime
print("This notebook was executed on: \n{}".format(datetime.datetime.now()))
%matplotlib inline
#%config InlineBackend.figure_format = 'svg'
import matplotlib.pyplot as plt
plt.rc('figure', figsize=(10, 6))
from collections import OrderedDict
import os
from astropy import units as u
from astropy.coordinates import SkyCoord
from astropy.table import Column, Table
import numpy as np
from herschelhelp_internal.flagging import gaia_flag_column
from herschelhelp_internal.masterlist import nb_astcor_diag_plot, remove_duplicates
from herschelhelp_internal.utils import astrometric_correction, mag_to_flux, flux_to_mag
OUT_DIR = os.environ.get('TMP_DIR', "./data_tmp")
try:
os.makedirs(OUT_DIR)
except FileExistsError:
pass
RA_COL = "kids_ra"
DEC_COL = "kids_dec"
###Output
_____no_output_____
###Markdown
I - Column selection
###Code
imported_columns = OrderedDict({
'ID': "kids_id",
'RAJ2000': "kids_ra",
'DECJ2000': "kids_dec",
'CLASS_STAR': "kids_stellarity",
'MAG_AUTO_U': "m_kids_u",
'MAGERR_AUTO_U': "merr_kids_u",
'MAG_AUTO_G': "m_kids_g",
'MAGERR_AUTO_G': "merr_kids_g",
'MAG_AUTO_R': "m_kids_r",
'MAGERR_AUTO_R': "merr_kids_r",
'MAG_AUTO_I': "m_kids_i",
'MAGERR_AUTO_I': "merr_kids_i",
'FLUX_APERCOR_10_U': "f_ap_kids_u",
'FLUXERR_APERCOR_10_U': "ferr_ap_kids_u",
'FLUX_APERCOR_10_G': "f_ap_kids_g",
'FLUXERR_APERCOR_10_G': "ferr_ap_kids_g",
'FLUX_APERCOR_10_R': "f_ap_kids_r",
'FLUXERR_APERCOR_10_R': "ferr_ap_kids_r",
'FLUX_APERCOR_10_I': "f_ap_kids_i",
'FLUXERR_APERCOR_10_I': "ferr_ap_kids_i"
})
catalogue = Table.read("../../dmu0/dmu0_KIDS/data/KIDS-DR3_HATLAS-SGP.fits")[list(imported_columns)]
for column in imported_columns:
catalogue[column].name = imported_columns[column]
epoch = 2014 #A range of observation dates from 2011 to 2015.
# Clean table metadata
catalogue.meta = None
# Adding flux and band-flag columns
for col in catalogue.colnames:
if col.startswith('m_'):
errcol = "merr{}".format(col[1:])
flux, error = mag_to_flux(np.array(catalogue[col]), np.array(catalogue[errcol]))
# Fluxes are added in µJy
catalogue.add_column(Column(flux * 1.e6, name="f{}".format(col[1:])))
catalogue.add_column(Column(error * 1.e6, name="f{}".format(errcol[1:])))
# Band-flag column
if "ap" not in col:
catalogue.add_column(Column(np.zeros(len(catalogue), dtype=bool), name="flag{}".format(col[1:])))
if col.startswith('f_'):
errcol = "ferr{}".format(col[1:])
#Convert fluxes in maggies to uJy
catalogue[col] *= 3631. * 1.e6
catalogue[col].unit = 'uJy'
catalogue[errcol] *= 3631. * 1.e6
catalogue[errcol].unit = 'uJy'
mag, mag_error = flux_to_mag(np.array(catalogue[col]) * 1.e-6,
np.array(catalogue[errcol]) * 1.e-6)
# Magnitudes are added
catalogue.add_column(Column(mag, name="m{}".format(col[1:])))
catalogue.add_column(Column(mag_error, name="m{}".format(errcol[1:])))
catalogue[:10].show_in_notebook()
###Output
_____no_output_____
###Markdown
II - Removal of duplicated sources We remove duplicated objects from the input catalogues.
###Code
SORT_COLS = ['merr_ap_kids_u',
'merr_ap_kids_g',
'merr_ap_kids_r',
'merr_ap_kids_i']
FLAG_NAME = 'kids_flag_cleaned'
nb_orig_sources = len(catalogue)
catalogue = remove_duplicates(catalogue, RA_COL, DEC_COL, sort_col=SORT_COLS,flag_name=FLAG_NAME)
nb_sources = len(catalogue)
print("The initial catalogue had {} sources.".format(nb_orig_sources))
print("The cleaned catalogue has {} sources ({} removed).".format(nb_sources, nb_orig_sources - nb_sources))
print("The cleaned catalogue has {} sources flagged as having been cleaned".format(np.sum(catalogue[FLAG_NAME])))
###Output
/opt/anaconda3/envs/herschelhelp_internal/lib/python3.6/site-packages/astropy/table/column.py:1096: MaskedArrayFutureWarning: setting an item on a masked array which has a shared mask will not copy the mask and also change the original mask array in the future.
Check the NumPy 1.11 release notes for more information.
ma.MaskedArray.__setitem__(self, index, value)
###Markdown
III - Astrometry correctionWe match the astrometry to the Gaia one. We limit the Gaia catalogue to sources with a g band flux between the 30th and the 70th percentile. Some quick tests show that this give the lower dispersion in the results.
###Code
gaia = Table.read("../../dmu0/dmu0_GAIA/data/GAIA_SGP.fits")
gaia_coords = SkyCoord(gaia['ra'], gaia['dec'])
nb_astcor_diag_plot(catalogue[RA_COL], catalogue[DEC_COL],
gaia_coords.ra, gaia_coords.dec, near_ra0=True)
delta_ra, delta_dec = astrometric_correction(
SkyCoord(catalogue[RA_COL], catalogue[DEC_COL]),
gaia_coords, near_ra0=True
)
print("RA correction: {}".format(delta_ra))
print("Dec correction: {}".format(delta_dec))
catalogue[RA_COL] += delta_ra.to(u.deg)
catalogue[DEC_COL] += delta_dec.to(u.deg)
nb_astcor_diag_plot(catalogue[RA_COL], catalogue[DEC_COL],
gaia_coords.ra, gaia_coords.dec, near_ra0=True)
###Output
_____no_output_____
###Markdown
IV - Flagging Gaia objects
###Code
catalogue.add_column(
gaia_flag_column(SkyCoord(catalogue[RA_COL], catalogue[DEC_COL]), epoch, gaia)
)
GAIA_FLAG_NAME = "kids_flag_gaia"
catalogue['flag_gaia'].name = GAIA_FLAG_NAME
print("{} sources flagged.".format(np.sum(catalogue[GAIA_FLAG_NAME] > 0)))
###Output
240146 sources flagged.
###Markdown
V - Flagging objects near bright stars VI - Saving to disk
###Code
catalogue.write("{}/KIDS.fits".format(OUT_DIR), overwrite=True)
###Output
_____no_output_____ |
src/main2/main/python/Notebooks/date_demo_rrule.ipynb | ###Markdown
Date Demo RruleShow how to use an rrule instance to make a custom date ticker - herewe put a tick mark on every 5th easterSee https://dateutil.readthedocs.io/en/stable/ for help with rrules
###Code
import matplotlib.pyplot as plt
from matplotlib.dates import (YEARLY, DateFormatter,
rrulewrapper, RRuleLocator, drange)
import numpy as np
import datetime
# Fixing random state for reproducibility
np.random.seed(19680801)
# tick every 5th easter
rule = rrulewrapper(YEARLY, byeaster=1, interval=5)
loc = RRuleLocator(rule)
formatter = DateFormatter('%m/%d/%y')
date1 = datetime.date(1952, 1, 1)
date2 = datetime.date(2004, 4, 12)
delta = datetime.timedelta(days=100)
dates = drange(date1, date2, delta)
s = np.random.rand(len(dates)) # make up some random y values
fig, ax = plt.subplots()
plt.plot_date(dates, s)
ax.xaxis.set_major_locator(loc)
ax.xaxis.set_major_formatter(formatter)
ax.xaxis.set_tick_params(rotation=30, labelsize=10)
plt.show()
###Output
_____no_output_____ |
EDA_Assignments/A_04_DataCleaningOutliers_en_SerhanOnerAksakal.ipynb | ###Markdown
Assignments for "Data Cleaning - Outliers" In this assignment, you continue to study the data of the `US Education System`. Again, please down it from here ([dataset](https://www.kaggle.com/noriuk/us-education-datasets-unification-project/home)). In this dataset, there is a lot of data on an annual basis. You can reach the explanations of this data from Kaggle again.First of all, apply the most appropriate one of the techniques for completing the missing values in your previous lesson. In order to answer the following questions, you must overcome the missing data.
###Code
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
df = pd.read_csv('states_all.csv')
df
df.info()
###Output
<class 'pandas.core.frame.DataFrame'>
RangeIndex: 1715 entries, 0 to 1714
Data columns (total 25 columns):
# Column Non-Null Count Dtype
--- ------ -------------- -----
0 PRIMARY_KEY 1715 non-null object
1 STATE 1715 non-null object
2 YEAR 1715 non-null int64
3 ENROLL 1224 non-null float64
4 TOTAL_REVENUE 1275 non-null float64
5 FEDERAL_REVENUE 1275 non-null float64
6 STATE_REVENUE 1275 non-null float64
7 LOCAL_REVENUE 1275 non-null float64
8 TOTAL_EXPENDITURE 1275 non-null float64
9 INSTRUCTION_EXPENDITURE 1275 non-null float64
10 SUPPORT_SERVICES_EXPENDITURE 1275 non-null float64
11 OTHER_EXPENDITURE 1224 non-null float64
12 CAPITAL_OUTLAY_EXPENDITURE 1275 non-null float64
13 GRADES_PK_G 1542 non-null float64
14 GRADES_KG_G 1632 non-null float64
15 GRADES_4_G 1632 non-null float64
16 GRADES_8_G 1632 non-null float64
17 GRADES_12_G 1632 non-null float64
18 GRADES_1_8_G 1020 non-null float64
19 GRADES_9_12_G 1071 non-null float64
20 GRADES_ALL_G 1632 non-null float64
21 AVG_MATH_4_SCORE 565 non-null float64
22 AVG_MATH_8_SCORE 602 non-null float64
23 AVG_READING_4_SCORE 650 non-null float64
24 AVG_READING_8_SCORE 562 non-null float64
dtypes: float64(22), int64(1), object(2)
memory usage: 335.1+ KB
###Markdown
**(1)** See `TOTAL_REVENUE`, which includes total revenue data, and `TOTAL_EXPENDITURE`, which includes total spending data. Do these variables contain outliers?
###Code
plt.figure(figsize=(12,8))
plt.subplot(1,2,1)
plt.boxplot(df.TOTAL_REVENUE.dropna())
plt.title('Total Revenue')
plt.subplot(1,2,2)
plt.boxplot(df.TOTAL_EXPENDITURE.dropna())
plt.title('Total Expenditure')
plt.show()
###Output
_____no_output_____
###Markdown
By using boxplot, we've seen that there are outliers in both data.Let us use histogram chart this time.
###Code
plt.figure(figsize=(12,8))
plt.subplot(1,2,1)
plt.hist(df.TOTAL_REVENUE.dropna())
plt.title('Total Revenue')
plt.subplot(1,2,2)
plt.hist(df.TOTAL_EXPENDITURE.dropna())
plt.title('Total Expenditure')
plt.show()
from scipy.stats import zscore
z_scores = zscore(df['TOTAL_REVENUE'].dropna())
for threshold in range(1,5):
print("Threshold value: {}".format(threshold))
print("Number of Outliers: {}".format(len((np.where(z_scores > threshold)[0]))))
print('------')
###Output
Threshold value: 1
Number of Outliers: 134
------
Threshold value: 2
Number of Outliers: 52
------
Threshold value: 3
Number of Outliers: 37
------
Threshold value: 4
Number of Outliers: 21
------
###Markdown
**(2)** If you find outliers in the total income `TOTAL_REVENUE` and total expenditure `TOTAL_EXPENDITURE` variables, eliminate these outliers by applying the techniques you learned in this lesson and verify that no outliers remain after doing this.
###Code
from scipy.stats.mstats import winsorize
winsorizing1 = winsorize(np.array(df.TOTAL_REVENUE.dropna()), (0.10, 0.15))
winsorizing1
from scipy.stats.mstats import winsorize
winsorizing2 = winsorize(np.array(df.TOTAL_EXPENDITURE.dropna()), (0.10, 0.15))
winsorizing2
np.percentile(df.TOTAL_REVENUE.dropna(),[10,90])
np.percentile(df.TOTAL_EXPENDITURE.dropna(),[10,90])
plt.title('Winsorized Total Revenue')
plt.boxplot(winsorizing1)
plt.show()
plt.boxplot(winsorizing2)
plt.title('Winsorized Total Expenditure')
plt.show()
plt.figure(figsize = (15,5))
plt.subplot(1,2,1)
plt.hist(df['TOTAL_REVENUE'].dropna())
plt.title('Total Revenue')
plt.subplot(1,2,2)
plt.hist(np.log(df['TOTAL_REVENUE'].dropna()))
plt.title("Total Revenue(log transformed)")
plt.show()
plt.figure(figsize = (15,5))
plt.subplot(1,2,1)
plt.hist(df['TOTAL_EXPENDITURE'].dropna())
plt.title('Total Expenditure')
plt.subplot(1,2,2)
plt.hist(np.log(df['TOTAL_EXPENDITURE'].dropna()))
plt.title("Total Expenditure(log transformed)")
plt.show()
###Output
_____no_output_____
###Markdown
**(3)** Subtract the total expenditure `TOTAL_EXPENDITURE` from the total income variable `TOTAL_REVENUE` to create a variable that can be considered as a budget deficit (without failing to contradict the outliers). Are there any outliers in this new variable? If any, clear these outliers.
###Code
budget_deficit = []
for value in range(0,1714):
budget_deficit.append( df.TOTAL_REVENUE[value] - df.TOTAL_EXPENDITURE[value] )
budget_deficit = pd.DataFrame(budget_deficit)
budget_deficit.columns = ['BUDGET_DEFICIT']
budget_deficit.head()
plt.figure(figsize= (8,8))
plt.boxplot(budget_deficit.BUDGET_DEFICIT.dropna())
plt.title('Budget Deficit')
plt.show()
winsorized_budget_deficit = winsorize(np.array(budget_deficit.BUDGET_DEFICIT.dropna()), (0.15, 0.10))
plt.boxplot(winsorized_budget_deficit)
plt.title('Winsorized Budget Deficit')
plt.show()
###Output
_____no_output_____
###Markdown
**(4)** Again create a variable that can be considered as a budget deficit by subtracting the total income `TOTAL_REVENUE` variable from the total expenditure `TOTAL_EXPENDITURE`. But this time, after clearing the outliers, do this. Are there any outliers in this new variable? It is useful to clean them, if any.
###Code
df.TOTAL_REVENUE.dropna()
df.TOTAL_EXPENDITURE.dropna()
budget_deficit_2 = []
for value in range(0,1274):
budget_deficit_2.append( df.TOTAL_REVENUE[value] - df.TOTAL_EXPENDITURE[value] )
budget_deficit_2 = pd.DataFrame(budget_deficit_2)
budget_deficit_2.columns = ['NEW_DEFICIT']
budget_deficit_2.head()
plt.figure(figsize=(8,8))
plt.boxplot(budget_deficit_2.NEW_DEFICIT)
plt.title('New Deficit')
plt.show()
new_winsorized_budget_deficit = winsorize(np.array(budget_deficit_2.NEW_DEFICIT.dropna()), (0.15, 0.10))
plt.boxplot(new_winsorized_budget_deficit)
plt.title('New Winsorized Budget Deficit')
plt.show()
###Output
_____no_output_____
###Markdown
**(5)** Now, we have two different budget variables that we have created in the third and fourth questions. Do you see the difference between these two?
###Code
plt.figure(figsize=(12,8))
plt.subplot(1,2,1)
plt.boxplot(budget_deficit.BUDGET_DEFICIT.dropna())
plt.title('Budget Deficit')
plt.subplot(1,2,2)
plt.boxplot(budget_deficit_2.NEW_DEFICIT)
plt.title('New Deficit')
plt.show()
plt.figure(figsize=(12,8))
plt.subplot(1,2,1)
plt.boxplot(winsorized_budget_deficit)
plt.title('Winsorized Budget Deficit')
plt.subplot(1,2,2)
plt.boxplot(new_winsorized_budget_deficit)
plt.title('New Winsorized Budget Deficit')
plt.show()
###Output
_____no_output_____
###Markdown
**I compared both the main and winsorized versions of what I've been given, but I couldn't see any difference. Actually, I thought there would be a difference. In my opinion, it is due to the my humanly-observations or techniques are not sufficient to observe what to get the difference.** **(6)** If the budget deficit was a very important variable for us, which method would be better to clear out contrary values. Is it the method you applied in the third question or the method in the fourth question?
###Code
"""
If I could've seen the difference, I'd have pick an option.
Anyway, my mind tells me that it is better to deal with the data AFTER dealing
with the missing values first.
"""
###Output
_____no_output_____ |
Breast_Cancer_Classification_CNN.ipynb | ###Markdown
###Code
# Hasil pengerjaan point 4.2
# Melakukan uji coba dari salah satu gambar test pada model 1 dan model 2 (10 point)
# Uji Coba Model 1
import numpy as np
from google.colab import files
from keras.preprocessing import image
import matplotlib.pyplot as plt
import matplotlib.image as mpimg
%matplotlib inline
uploaded = files.upload()
for fn in uploaded.keys():
#predicting images
path = fn
img = image.load_img(path, target_size=(250,250))
imgplot = plt.imshow(img)
x = image.img_to_array(img)
x = np.expand_dims(x, axis = 0)
images = np.vstack([x])
classes = model1.predict(images, batch_size=10)
classed = model2.predict(images, batch_size=10)
if classes == 0:
print('SC1 : Benign')
else:
print('SC1 : Malignant')
if classed == 0:
print('SC2 : Benign')
else:
print('Sc2 : Malignant')
# Hasil pengerjaan point 4.3
# Klasifikasi report dari SC1 (5 point)
from sklearn.metrics import classification_report
pred = model1.predict(x_test)
labels = (pred > 0.5).astype(np.int)
print(classification_report(y_test, labels))
# Hasil pengerjaan point 4.3
# Klasifikasi report dari SC2 (5 point)
from sklearn.metrics import classification_report
pred = model2.predict(x_test)
labels = (pred > 0.5).astype(np.int)
print(classification_report(y_test, labels))
###Output
precision recall f1-score support
0 0.82 0.73 0.77 176
1 0.88 0.92 0.90 369
accuracy 0.86 545
macro avg 0.85 0.83 0.84 545
weighted avg 0.86 0.86 0.86 545
|
InterviewCake Questions.ipynb | ###Markdown
You have a list of integers, and for each index you want to find the product of every integer except the integer at that index.Write a function get_products_of_all_ints_except_at_index() that takes a list of integers and returns a list of the products.For example, given: `[1, 7, 3, 4]`your function would return: ` [84, 12, 28, 21]`by calculating: `[7 * 3 * 4, 1 * 3 * 4, 1 * 7 * 4, 1 * 7 * 3]`Do not use division in your solution.
###Code
from functools import reduce
def get_products_of_all_ints_except_at_index(arr):
results = []
if len(arr) < 2:
raise Exception("Arrays too short, can't do it")
for index, value in enumerate(arr):
new_array = arr[0:index] + arr[index+1:]
product = reduce((lambda x, y: x * y), new_array)
results.append(product)
return results
arr = [1, 7, 3, 4]
print(get_products_of_all_ints_except_at_index(arr))
# arr = [1]
# print(get_products_of_all_ints_except_at_index(arr))
arr = [1, 2]
print(get_products_of_all_ints_except_at_index(arr))
###Output
[84, 12, 28, 21]
[2, 1]
###Markdown
Apple StocksWrite an efficient function that takes stock_prices_yesterday and returns the best profit I could have made from 1 purchase and 1 sale of 1 Apple stock yesterday.
###Code
from IPython.core.display import Image, display
from IPython.display import Image, Markdown
import random
def get_max_profit(stock_prices):
'''returns the best profit I could have made from 1 purchase and 1 sale of 1 Apple stock yesterday.
have to buy before selling
O(n^2) Solution
'''
max_profit = 0
for inner_index in range(len(stock_prices)):
for outer_index in range(len(stock_prices)):
earlier_time = min(inner_index, outer_index)
later_time = max(inner_index, outer_index)
# Get the earlier/later prices for correct ordering
earlier_price = stock_prices[earlier_time]
later_price = stock_prices[later_time]
potential_profit = later_price - earlier_price
max_profit = max(max_profit, potential_profit)
return max_profit
stock_prices_yesterday = []
print(get_max_profit(stock_prices_yesterday) == 0)
stock_prices_yesterday = [6]
print(get_max_profit(stock_prices_yesterday) == 0)
stock_prices_yesterday = [10, 7, 5, 8, 11, 9]
print(get_max_profit(stock_prices_yesterday) == 6)
def get_max_profit(stock_prices):
'''returns the best profit I could have made from 1 purchase and 1 sale of 1 Apple stock yesterday.
have to buy before selling
O(n^2) Solution
'''
max_profit = 0
for inner_index in range(len(stock_prices)):
for outer_index in range(len(stock_prices[inner_index:])):
earlier_time = min(inner_index, outer_index)
later_time = max(inner_index, outer_index)
# Get the earlier/later prices for correct ordering
earlier_price = stock_prices[earlier_time]
later_price = stock_prices[later_time]
potential_profit = later_price - earlier_price
max_profit = max(max_profit, potential_profit)
return max_profit
stock_prices_yesterday = []
print(get_max_profit(stock_prices_yesterday) == 0)
stock_prices_yesterday = [6]
print(get_max_profit(stock_prices_yesterday) == 0)
stock_prices_yesterday = [10, 7, 5, 8, 11, 9]
print(get_max_profit(stock_prices_yesterday) == 6) #incorrect
###Output
True
True
False
###Markdown
Need to do better than O(n^2), so it'll probably be either O(n log n) or O(n). Let's try a greedy approach.
###Code
def get_max_profit(stock_prices):
'''returns the best profit I could have made from 1 purchase and 1 sale of 1 Apple stock yesterday.
have to buy before selling.
Came up with this on my own! Runtime is O(n)
'''
if len(stock_prices) < 2:
return 0
min_buy = stock_prices[0]
max_sell = stock_prices[1]
print(min_buy, max_sell)
for time in range(len(stock_prices)-1):
if time == 0:
continue
buy = stock_prices[time]
sell = stock_prices[time+1]
min_buy = min(min_buy, buy)
max_sell = max(max_sell, sell)
print(min_buy, max_sell)
return max_sell - min_buy
# stock_prices_yesterday = []
# print(get_max_profit(stock_prices_yesterday) == 0)
# stock_prices_yesterday = [6]
# print(get_max_profit(stock_prices_yesterday) == 0)
stock_prices_yesterday = [10, 7, 5, 8, 11, 9]
print(get_max_profit(stock_prices_yesterday))
# stock_prices_yesterday = [4, 3, 2, 1]
# print(get_max_profit(stock_prices_yesterday))
# stock_prices_yesterday = [4, 4, 4, 4]
# print(get_max_profit(stock_prices_yesterday))
def get_max_profit(stock_prices_yesterday):
# make sure we have at least 2 prices
if len(stock_prices_yesterday) < 2:
raise IndexError('Getting a profit requires at least 2 prices')
# we'll greedily update min_price and max_profit, so we initialize
# them to the first price and the first possible profit
min_price = stock_prices_yesterday[0]
max_profit = stock_prices_yesterday[1] - stock_prices_yesterday[0]
for index, current_price in enumerate(stock_prices_yesterday):
# skip the first (0th) time
# we can't sell at the first time, since we must buy first,
# and we can't buy and sell at the same time!
# if we took this out, we'd try to buy *and* sell at time 0.
# this would give a profit of 0, which is a problem if our
# max_profit is supposed to be *negative*--we'd return 0.
if index == 0:
continue
# see what our profit would be if we bought at the
# min price and sold at the current price
potential_profit = current_price - min_price
# update max_profit if we can do better
max_profit = max(max_profit, potential_profit)
# update min_price so it's always
# the lowest price we've seen so far
min_price = min(min_price, current_price)
return max_profit
stock_prices_yesterday = [10, 7, 5, 8, 11, 9]
print(get_max_profit(stock_prices_yesterday))
stock_prices_yesterday = [4, 3, 2, 1]
print(get_max_profit(stock_prices_yesterday))
stock_prices_yesterday = [4, 4, 4, 4]
print(get_max_profit(stock_prices_yesterday))
###Output
6
-1
0
|
_sources/curriculum-notebooks/Arts/MusicMathematicsOfPitch/music-mathematics-of-pitch.ipynb | ###Markdown
 Music - Mathematics of PitchThere are many connections between mathematics and music, ranging from how rhythms fit together, to how notes fit together in chords, to the structure of counterpoint, and more. We will focus on the connection between the pitch of notes and fractions. We will see that the idea of harmonious notes is closely connected to simplicity in fractions. We will also look at how a problem with these fractions was solved in the Baroque period by creating a well-tempered scale that made singers and instruments sound harmonious in all possible key signatures.
###Code
%matplotlib inline
from matplotlib.pyplot import *
from numpy import * ## for numerical functions
from math import tau
from IPython.display import Audio, display, HTML ## to output audio
from ipywidgets import interact, RadioButtons
HTML('''<script>
function code_toggle() {
if (code_shown){
$('div.input').hide('500');
$('#toggleButton').val('Show Code')
} else {
$('div.input').show('500');
$('#toggleButton').val('Hide Code')
}
code_shown = !code_shown
}
$( document ).ready(function(){
code_shown=false;
$('div.input').hide()
});
</script>
<form action="javascript:code_toggle()"><input type="submit" id="toggleButton" value="Show Code"></form>''')#
###Output
_____no_output_____
###Markdown
OutlineA quick overview of what will be covered in this notebook.- First, we test the sound system on your computer- Then we briefly discuss some of the characteristics of musical notes, including: - loudness - duration - pitch - timbre - envelope- We then look at the history of finding notes in a scale, using simple fractions- The Circle of Fifths method is discussed- We then look at the well-tempered tuning, which solved an important problem for musicians.Throughout we will use code to generate synthetic sounds on the computer to demonstrate many of these ideas. Setting upThis notebook will play sound through the speakers or headphones of your computer. To make sure this works, try clicking on the arrow of the audio icon below. You should hear some random noise.If you don't hear any sound at all, or it's too loud, try adjusting the volume and making sure that the audio devices are activated on your computer.Once you can get sound from the audio icon below, please continue to the notebook content below.
###Code
Fs = 44100
random_sd = random.randn(Fs)
display("Click on the arrow to test sound. ")
Audio(data=random_sd, rate=Fs)
###Output
_____no_output_____
###Markdown
Notes of the musical scaleMusic is make up of individual sounds often refered to as "notes". In the movie "The Sound of Music," the children are taught a song that names the individual notes, as in these lyrics:```Do-re-mi-fa-so-la-ti-doDoe, a deer, a female deerRay, a drop of golden sunMe, a name I call myselfFar, a long, long way to runSew, a needle pulling threadLa, a note to follow SewTea, a drink with jam and breadThat will bring us back to Doe.```If you haven't seen the movie, here is a clip from YouTube:
###Code
%%html
<iframe width="560" height="315" src="https://www.youtube.com/embed/pLm07s8fnzM" frameborder="0" allow="accelerometer; autoplay; encrypted-media; gyroscope; picture-in-picture" allowfullscreen></iframe>
###Output
_____no_output_____
###Markdown
The note names Do, Re, Mi, ... were originally Ut, Re, Mi, Fa, So, La, Si, ... and came from a hymn in Latin, circa the year 1000. The first stanza is:```Ut queant laxisresonare fibris,Mira gestorumfamuli tuorum,Solve pollutilabii reatum,Sancte Iohannes.```and the first two letters of each line gave the names of the notes. Except for the initials SI in the last line, for Saint Iohannes. The word "Ut" was eventually replaced with "Do" for Dominus. (of course)So there's a little Latin for you!Nowadays we usually refer to the notes in the scale by the letters C,D,E,F,G,A,B and return to C. These correspond to the white keys on the piano, while sharps and flats are the black keys. Characteristics of notes in musicNotes have a number of characteristics, including the loudness, the duration, the pitch, timbre, and envelope. Perhaps you already know what these mean: certainly the loudness and duration, or length in time, should be clear. The pitch is basically whether a note sounds high (like a young child's voice) or low (like a man's voice). Timbre characterizes the difference between, say, a flute and an electric guitar, when the notes from the flute are very clean and pure sounding, while an electric guitar can sound harsh and rough. The envelope describes how the note evolves over time: does it have a sharp initial attack, is it sustained for a long period, does it decay slowly to silence or stop abruptly.Here are five different examples of sounds which vary the individual characteristics of the notes: loudness, duration, pitch, timbre, and envelope. Real sounds of course can vary much more than these synthetic notes. Click on the "Play" button (the arrow head) to hear each one.
###Code
def heaviDecay(t):
return heaviside(t,1)*exp(-t)
Fs = 44100
Len = 3
t = linspace(0,Len,Len*Fs)
freq = 261.6 # middle C
sineWave = sin(tau*freq*t)
intSteps = floor(1+4*t/Len)
varyLoudness = intSteps**2*sineWave
varyDuration = maximum(0,sin(tau*4*(t/Len)**2))**.5*sineWave
varyPitch = sin(tau*intSteps*freq*t)
varyTimbre = maximum(-1,minimum(1,intSteps**2*sineWave))
varyEnvelope = (heaviDecay(10*t)+heaviDecay(5*(t-1))+heaviDecay(t-2))*sineWave
a1=Audio(data=varyLoudness, rate=Fs)
a2=Audio(data=varyDuration, rate=Fs)
a3=Audio(data=varyPitch, rate=Fs)
a4=Audio(data=varyTimbre, rate=Fs)
a5=Audio(data=varyEnvelope, rate=Fs)
display("A sound with changing loudness.",a1,
"Several notes with changing duration.",a2,
"A sound with changing pitch.",a3,
"A sound with changing timbre",a4,
"Several notes with different envelopes.",a5)
###Output
_____no_output_____
###Markdown
Sample notesOf course, when creating a note, there is the possiblity of changing any or all of these characteristics. For instance, by adjusting these there sliders, you can alter the pitch, timbre and envelope of a note and hear what it sounds like.Try it out now: click on the sliders to move the buttons and hear the resulting sound.
###Code
## Code block E
Fs = 44100
Len3 = 3
t3 = linspace(0,Len3,Len3*Fs)
freq_c = 261.6 # middle C
sineWave = sin(tau*freq_c*t3)
intSteps = floor(1+4*t3/Len3)
run_e = 0
def play_e(pitch,timbre,envelope):
global run_e
if run_e == 0:
run_e = 1
return
data1 = heaviDecay(envelope*t3)*maximum(-1,minimum(1,timbre**2*sin(tau*pitch*t3)))
a1=Audio(data=data1, rate=Fs,autoplay=True)
display(a1)
interact(play_e,pitch=(200,400),timbre=(1,5),envelope=(1,10));
###Output
_____no_output_____
###Markdown
Pitch and harmonyPeople in ancient civilizations noticed a long time ago that notes of certain pitches sound "good" together. We often say such notes sound harmonious. They also noticed that the pitch of sound produced by singers, or instruments, were somehow related to the size, shape and weight of the singer or instrument. For instance, a big man would typically sing in a low pitch, while a small woman or child would sing in a higher pitch. A large drum makes a deep, low sound while a small drum or wood block would make a high sound. Pitch is related to the rate of vibration of an object producing sounds. Although the ancients could not measure this directly, certainly they would have noticed by touching their throats while singing, that a low-pitched sound has a slow vibration, and a high-pitched sound has a faster vibration. We can now measure the frequency of notes, and discover that the range of human voices produce vibrations with frequency as low as 80 cycles per second (for a male bass singer) to as high as 1000 cycles per second (for a female soprano singer). Try this out with the slide below. Perhaps you can sing along to some of the notes!
###Code
## Code block F
Fs = 44100
Len3 = 3
t3 = linspace(0,Len3,Len3*Fs)
run_f = 0
def play_f(pitch):
global run_f
if run_f == 0:
run_f = 1
return
a1=Audio(data=sin(tau*pitch*t3), rate=Fs,autoplay=True)
display(a1)
interact(play_f,pitch=(80,1000));
###Output
_____no_output_____
###Markdown
Harmony and arithmeticIt was perhaps the ancient Greeks who first noticed a connection between harmonious notes and mathematics. They observed that strings of different lengths, but the same thickness and tension, would often produce harmonious pairs of tones if the string lengths were in the ratio of simple fractions. You may have noticed this yourself if you play a guitar or ukelele. For instance, one string that was half the length of another would produce a note that was an octave higher that the other string. A string that was two-thirds the length would produce the C-to-G interval, which we now call "a fifth." The Greeks didn't know it, but the frequency (or pitch) was directly related to the reciprocal of these fractions. So the string of one-half (1/2) length would have 2 times the frequency. The string of two-thirds (2/3) length would have three-halves (3/2) the frequency. With this idea in mind, we can take a few fractions with a small denominator like 4, and get some really nice notes.Indeed, the fractions 4/4, 5/4, 6/4, 7/8 and 8/4 correspond to frequencies of the main notes in a Boogie-Woogie chord. In our modern notation, these are the notes C,E,G,B-flat and the C an octave higher.We can create these notes synthetically on the computer very easily. Try clicking on the controls below to hear these familiar notes. The relevant fraction is shown below, and is precisely the fraction we used to create the note.
###Code
## Codeblock A
Fs = 44100
Len05 = 0.5
t05 = linspace(0,Len05,int(Len05*Fs))
freq_c = 261.6 # middle C
notes_a = ['C (1)', 'E (5/4)', 'G (6/4)', 'B flat (7/4)','C (2)' ]
fracs_a = array([1,5/4,3/2,7/4,2])
run_a = 0
def play_a(Note):
global run_a
if run_a == 0:
run_a = 1
return
i = notes_a.index(Note)
frac = fracs_a[i]
a1=Audio(data=sin(frac*tau*freq_c*t05)*heaviDecay(4*t05), rate=Fs,autoplay=True)
display(Note,a1)
interact(play_a,Note=RadioButtons(options=notes_a,value=notes_a[-1]));
###Output
_____no_output_____
###Markdown
Scales and fractionsWe can go further and create the full scale from C to C, using the small fractions 1, 9/8, 5/4, 4/3, 3/2, 5/3, 15/8, and 2. Granted, the fractions 9/8 and 15/8 stand out as a bit unusual, as they seem to have a pretty big denominator -- but these are the fractions that give us the familiar Western scale. Below, you can click on the radio buttons to see what those notes sound like.
###Code
## Codeblock B
Fs = 44100
Len05 = .5
t05 = linspace(0,Len05,int(Len05*Fs))
freq_c = 261.6 # middle C
notes_b = ['C (1)', 'D (9/8)', 'E (5/4)', 'F (4/3)', 'G (3/2)', 'A (5/3)', 'B (15/8)', 'C (2)' ]
fracs_b = array([1,9/8,5/4,4/3,3/2,5/3,15/8,16/8])
run_b = 0
def play_b(Note):
global run_b
if run_b == 0:
run_b = 1
return
i = notes_b.index(Note)
frac = fracs_b[i]
a1=Audio(data=sin(frac*tau*freq_c*t05)*heaviDecay(4*t05), rate=Fs,autoplay=True)
display(Note,a1)
interact(play_b,Note=RadioButtons(options=notes_b,value=notes_b[-1]));
###Output
_____no_output_____
###Markdown
Out of tuneDo those notes sound right to you? If you are an experienced musician, they might seem a little off. In fact, there is a problem with these notes. Although they are designed to be harmonious (as the ancient Greeks felt they are), there is a problem when a musician starts to transpose notes to different keys. The problem is when you shift all those fractions up by a fixed amount, the new frequencies don't necessarily align with the old.For instance, if we take the C scale and shift to the G scale, we should take the fractions 1, 9/8, 5/4, 4/3, ... and multiply them all by 3/2 (making the base frequency 3/2). We end up with the fractions$$ \frac{3}{2}\times\left[1, \frac{9}{8}, \frac{5}{4}, \frac{4}{3}, \frac{3}{2}, \frac{5}{3}, \frac{15}{8}, 2\right] = \left[\frac{3}{2}, \frac{27}{16}, \frac{15}{8}, 2, \frac{9}{4}, \frac{15}{6}, \frac{45}{16}, 3\right].$$Now, on the right-hand side, we see the familiar fractions 3/2, 15/8 and 2. But in the middle is that 27/16 where we should be expecting a 5/3, corresponding to the A in our scale. It is out of tune, by quite a bit, because$$ \frac{5}{3} = 1.6667 \neq 1.6875 = \frac{27}{16}.$$So this note might sound terribly out of tune. Perhaps this is most easily illustrated with the Circle of Fifths idea. Circle of FifthsHere is an interesting method for creating the notes in our Western scales. The idea is that the interval C to G, can be used to generate all the notes in the Western tonal scale.Graphically, we can look at the following picture, which lists all the notes C,D,E,F,G, A, and B, as well as the sharps that can occur. Note that sharps correspond to the black keys on the piano. The Circle of Fifths. The interval from C to G is called a "fifth" in western music, because G is the fifth note in the usual diatonic scale that goes C,D,E,F,G,A,B,C. This fifth is marked on the above circle by the blue arc going from C to G.We can also get from C to G on this circle by counting off "ticks" on the circle: it takes 7 ticks to go clockwise from C to G. Each tick corresponds to a semitone in music, so there are seven semitones in a fifth. If you count another seven ticks from G, it will bring you past the C at the top of the circle and get you to D. This is marked by the second arc, going from G to D. Exercise:1. Continue this pattern. Count seven ticks clockwise from D, you should end up at A. Repeat the seven ticks: what comes after A? Then what comes after that?2. Repeat this pattern. After a total of 12 repeats, you should come back to the beginning note. What is the seuqence of notes generated by following the circle of fifths? (Note: We saw above that the sequence starts as C, G, D, A, ...)3. Did you notice that the sharp notes all come at the end of the sequence? Why is that?4. Draw your own circle of fifths, and include all 12 arcs that move you across all the notes in the circle.5. The **Circle of Fourths**. The interval C to F is called a fourth, and consists of 5 semitones (moving clockwise by 5 ticks on the circle). Check yourself that by moving through a fourth each time in the circle, you will eventually pass through every note on the circle. Mathematics in the Circle of Fifths.We discussed earlier that the jump from C to G corresponds to increasing the frequency of our note by a factor 3/2. This is true for any leap of a fifth: the frequencies increase by a factor of 3/2. So, if we think of 1 as our base frequency for C, then 3/2 is the frequency for G, and (3/2)x(3/2) = 9/4 should correspond to the note D. But of course, this D is too high -- it is an octave higher than what we want, so divide by 2 to bring it down an octave, to get (9/4)/2 = 9/8. This agrees with our fractional scales discussed above.To go from D to A, we again multiply by 3/2, so (9/8)x(3/2) = 27/16. This is not quite 5/3 = 1.66667 but pretty close, as 27/16 = 1.6875. From A to E, we multiply again by 3/2 to get 81/32 and again this is too high by an octave, so we divide by 2 to get $$81/64 \approx 80/64 = 5/4.$$We should be able to continue with these fractions to get all possible frequencies for the notes on the circle. Unfortunately, this is not quite right, as we will see in the next section. Well-tempered tuning and roots of twoThe problem with the fractional tuning is this Circle of Fifths doesn't bring you back to C, exactly. The math behind this is that each time you move by a fifth, the frequency will increase by exactly 3/2. So the frequencies increase like this pattern:$$ 1, (3/2), (3/2)^2, (3/2)^3, \ldots, (3/2)^{12}.$$But we can compute $(3/2)^{12} = 129.746$. This is just a bit bigger than $128 = 2^7$, which is a power of two. Remember, powers of 2 give us octave jumps. So the circle of fifth brings us to a note slightly higher than the C which is seven octaves higher. This got to be such a problem for musicians that in Baroque period, various people proposed a "well-tempered" system of tuning for notes where the intervals for frequencies differences are given by powers of the number 2.You may have heard of Bach's composition "The Well Tempered Clavier" which is a collection of 24 pieces in all major and minor keys, demonstrating the utility of the well-tempering system.
###Code
%%html
<iframe width="560" height="315" src="https://www.youtube.com/embed/ezZdbzreNcs" frameborder="0" allow="accelerometer; autoplay; encrypted-media; gyroscope; picture-in-picture" allowfullscreen></iframe>
###Output
_____no_output_____
###Markdown
Fixing the mathThe basic idea in well-tempering is to choose an number close to 3/2 = 1.5 so that its twelfth power is exactly 128. In fact, we find$$ 1.4983071^{12} = 128.$$ Now when we go around the circle of fifths, we will come back exactly to C, in exact frequencies. If you know a bit of algebra, you might recognize this number 1.4983071 as the 12-th root of 128. Another way to describe the circle of fifths is to measure the size of the semitone. We need a number that solves $$x^{12} = 2$$which just says we need 12 semitones in an octave. The answer is the twelfth root of two, or approximately$$ x= 1.0594631.$$Now this has solved our problem with tuning. All our notes are related to each other by powers of 2. Shifting up and down the scale amounts to multiplying or dividing by some power of two, so every notes stays in the proper relationship to other notes. There are distinct 12 notes in this system. If we play them all, we get the chromatic scale which includes the notes- C, C, D, D, E, F, F, G, G, A, A, B, and back to C.You might well ask, have we lost the exact harmony of the ancient Greeks? Well, indeed we have, but only approximately. See if you can hear the difference, by playing these well-tempered notes in the major C scale. Click on the radio buttons to hear the notes.
###Code
## Code block C
Fs = 44100
Len05 = .5
t05 = linspace(0,Len05,int(Len05*Fs))
freq_c = 261.6 # middle C
notes_c = ['C (1.0)', 'D (1.0595)^2', 'E (1.0595)^4', 'F (1.0595)^5', 'G (1.0595)^7', 'A (1.0595)^9', 'B (1.0595)^11', 'C (2.0)' ]
fracs_c = (2**(1/12))**(array([0,2,4,5,7,9,11,12]))
run_c = 0
def play_c(Note):
global run_c
if run_c == 0:
run_c = 1
return
i = notes_c.index(Note)
frac = fracs_c[i]
a1=Audio(data=sin(frac*tau*freq_c*t05)*heaviDecay(4*t05), rate=Fs,autoplay=True)
display(Note,a1)
interact(play_c,Note=RadioButtons(options=notes_c,value=notes_c[-1]));
###Output
_____no_output_____
###Markdown
Side by side comparisonHere we have both the fractional scale, and the well-tempered scale.Click on the radio buttons, to see if you can hear the difference. Notice the display shows the exact value of the fraction and the power of two. In all cases, the difference is less than a percent, which is very difficult to hear unless you are a trained musician. (The A and the E sort of stand out as quite different.)
###Code
## Code block G
Fs = 44100
Len05 = .5
t05 = linspace(0,Len05,int(Len05*Fs))
freq_c = 261.6 # middle C
notes_g = notes_b+notes_c
fracs_g = append(fracs_b,fracs_c)
run_g = 0
def play_g(Note):
global run_g
if run_g == 0:
run_g = 1
return
i = notes_g.index(Note)
frac = fracs_g[i]
a1=Audio(data=sin(frac*tau*freq_c*t05)*heaviDecay(4*t05), rate=Fs,autoplay=True)
display("Note "+Note,"Decimal value: {:.6f}".format(frac), a1)
interact(play_g,Note=RadioButtons(options=notes_g,value=notes_g[-1]));
###Output
_____no_output_____ |
Batch 7 Day 3 Assignment.ipynb | ###Markdown
Assignment 1
###Code
i = int( input("Enter the Aircraft altitude"))
if i <= 1000:
print("Proceed to land your aircraft")
elif i > 1000 and i <= 4500:
print("Bring down your aircraft to 1000ft")
elif i > 4500 and i <= 6500:
print ("Please turn around!")
###Output
_____no_output_____
###Markdown
Assignment 2
###Code
for i in range(1,201):
if i < 1:
print(i,"is not a prime number")
else:
for j in range(2,i):
if (i % j) == 0:
print (i, "is a prime number")
else:
print (i,"is not a prime number")
###Output
_____no_output_____ |
Codes/.ipynb_checkpoints/climate_starter-checkpoint.ipynb | ###Markdown
Reflect Tables into SQLAlchemy ORM
###Code
# Python SQL toolkit and Object Relational Mapper
import sqlalchemy
from sqlalchemy.ext.automap import automap_base
from sqlalchemy.orm import Session
from sqlalchemy import create_engine, func, inspect
# create engine to hawaii.sqlite
engine = create_engine("sqlite:///hawaii.sqlite")
# reflect an existing database into a new model
Base = automap_base()
# reflect the tables
Base.prepare(engine, reflect=True)
# View all of the classes that automap found
Base.classes.keys()
# Save references to each table
# Create our session (link) from Python to the DB
session = Session(bind=engine)
###Output
_____no_output_____
###Markdown
Exploratory Precipitation Analysis
###Code
# Find the most recent date in the data set.
recent_date = session.query()
# Design a query to retrieve the last 12 months of precipitation data and plot the results.
# Starting from the most recent data point in the database.
# Calculate the date one year from the last date in data set.
# Perform a query to retrieve the data and precipitation scores
# Save the query results as a Pandas DataFrame and set the index to the date column
# Sort the dataframe by date
# Use Pandas Plotting with Matplotlib to plot the data
# Use Pandas to calcualte the summary statistics for the precipitation data
###Output
_____no_output_____
###Markdown
Exploratory Station Analysis
###Code
# Design a query to calculate the total number stations in the dataset
# Design a query to find the most active stations (i.e. what stations have the most rows?)
# List the stations and the counts in descending order.
# Using the most active station id from the previous query, calculate the lowest, highest, and average temperature.
# Using the most active station id
# Query the last 12 months of temperature observation data for this station and plot the results as a histogram
###Output
_____no_output_____
###Markdown
Close session
###Code
# Close Session
session.close()
###Output
_____no_output_____ |
notebooks/curve_interface_average_structure.ipynb | ###Markdown
Part 0: Initialize
###Code
host = 'atat_21mer'
a_agent = AvgAgent(findhelix_folder, host)
###Output
/home/yizaochen/codes/dna_rna/length_effect/find_helical_axis/atat_21mer/input exists
/home/yizaochen/codes/dna_rna/length_effect/find_helical_axis/atat_21mer/avg_structure exists
###Markdown
Part 1: Convert avg.crd to avg.pdb
###Code
a_agent.convert_crd_to_pdb()
###Output
Convert /home/yizaochen/codes/dna_rna/all_systems/atat_21mer/bdna+bdna/input/heavyatoms/bdna+bdna.nohydrogen.avg.crd to /home/yizaochen/codes/dna_rna/all_systems/atat_21mer/bdna+bdna/input/heavyatoms/bdna+bdna.nohydrogen.avg.pdb
Check by:
vmd -pdb /home/yizaochen/codes/dna_rna/all_systems/atat_21mer/bdna+bdna/input/heavyatoms/bdna+bdna.nohydrogen.avg.pdb
###Markdown
Part 2: Change B-chain ID from 1-21 to 22-42
###Code
# check pdb, to see whether require to change resid
cmd = f'vim {a_agent.avg_pdb}'
print(cmd)
a_agent.backup_avg_pdb()
change = True # True or False
if change:
a_agent.change_resid_to_modi()
###Output
Write PDB: /home/yizaochen/codes/dna_rna/length_effect/find_helical_axis/tgtg_21mer/input/bdna_modi_avg.pdb
###Markdown
Part 3: Execute Curves+ and find helix
###Code
a_agent.curveplus_find_haxis()
###Output
Write PDB: /home/yizaochen/codes/dna_rna/length_effect/find_helical_axis/tgtg_21mer/avg_structure/haxis.avg.pdb
cp /home/yizaochen/codes/dna_rna/length_effect/find_helical_axis/tgtg_21mer/r+bdna_X.pdb /home/yizaochen/codes/dna_rna/length_effect/find_helical_axis/tgtg_21mer/avg_structure/haxis.smooth.avg.pdb
###Markdown
Part 4: Use VMD to show
###Code
a_agent.vmd_check()
###Output
cd /home/yizaochen/codes/bentdna
vmd -pdb /home/yizaochen/codes/dna_rna/all_systems/tgtg_21mer/bdna+bdna/input/heavyatoms/bdna+bdna.nohydrogen.avg.pdb
mol new /home/yizaochen/codes/dna_rna/length_effect/find_helical_axis/tgtg_21mer/avg_structure/haxis.avg.pdb type pdb
mol new /home/yizaochen/codes/dna_rna/length_effect/find_helical_axis/tgtg_21mer/avg_structure/haxis.smooth.avg.pdb type pdb
source ./tcl/draw_aa_haxis.tcl
|
notebook/ldn_sport_participation.ipynb | ###Markdown
Plot % of zero activity per borough/region
###Code
do_all = False
with sns.axes_style('whitegrid'):
for area in areas:
if not do_all and area != 'Southwark':
continue
fig, ax = plt.subplots(figsize=(11, 7), nrows=1, ncols=1)
df_area = df_zero[df_zero['area'] == area]
if len(df_area) > 0:
df_area.dropna()['percentage'].plot(ax=ax)
ax.yaxis.set_major_formatter(FuncFormatter(lambda y, _: '{:.2%}'.format(y)))
ax.legend(loc='lower left')
ax.set_title(area)
plt.tight_layout()
plt.show()
###Output
_____no_output_____
###Markdown
Curvature index**Goal**: to be able to compare boroughs in terms of the impact of the Olympics on the sport participation; ideally, produce a single number to quantify the impact and its durability.**Methodology**:For many boroughs, it seems the year 2011-2012 witnessed a decrease in the 'zero' population, i.e a larger fraction of people exercised at least once in the week.This surge in sport activity seems to have reverted in the years after.We compute a curvature index:* ((mean of 'zero' % after - 'zero' % @2011-2012) - ('zero' % @2011-2012 - mean of 'zero' % before)) / 2In case of transient impact, the curvature at 2011-2012 should be high, indicating a peak in sport participation that quickly reverted.**Results**:The year 2012 corresponds indeed to a peak in sport participation, most likely driven by renewed interest in sports at the time of the Olympics and possibly local policies to promote sport and sport infrastructure. The curvature index is positive for many boroughs, indicating a transient impact (for instance the pro-sport policies may have only lasted for the summer 2012).
###Code
sport_min_year = dict()
# sport_slope = dict()
sport_curvature = dict()
for area in areas:
df_area = df_zero[df_zero['area'] == area]
if len(df_area) > 0:
sport_min_year[area] = df_area['percentage'].idxmin()
# sport_slope[area] = (df_area.iloc[:6].mean()['percentage'] - df_area.iloc[5:].mean()['percentage'])
sport_curvature[area] = (
(df_area.iloc[6:].mean()['percentage'] - df_area.iloc[5]['percentage']) - (df_area.iloc[5]['percentage'] - df_area.iloc[:5].mean()['percentage'])
) / 2
sport_min_year = pd.Series(sport_min_year).sort_values()
# sport_slope = pd.Series(sport_slope).sort_values()
sport_curvature = pd.Series(sport_curvature).sort_values()
with sns.axes_style('whitegrid'):
fig, axes = plt.subplots(figsize=(11, 14), nrows=2, ncols=1)
ax = axes[0]
sport_min_year.hist(ax=ax, bins=len(years))
ax.set_title('Sport Best Year')
# ax = axes[1]
# sport_slope.plot.bar(ax=ax)
# ax.set_title('Sport Slope Index')
ax = axes[1]
sport_curvature.plot.bar(ax=ax)
ax.set_title('Sport Curvature Index')
plt.tight_layout()
plt.show()
###Output
_____no_output_____
###Markdown
Total plot
###Code
with sns.axes_style('whitegrid'):
fig, ax = plt.subplots(figsize=(11, 7), nrows=1, ncols=1)
# df_zero[df_zero['area'] == 'Southwark'].dropna().sort_values('year')[['year', 'percentage']].set_index('year').plot(ax=ax)
df_zero.groupby('year').mean().sort_values('year')['percentage'].plot(ax=ax, color='r', label='zero')
df_one.groupby('year').mean().sort_values('year')['percentage'].plot(ax=ax, color='b', label='one+')
df_three.groupby('year').mean().sort_values('year')['percentage'].plot(ax=ax, color='g', label='three')
ax.yaxis.set_major_formatter(FuncFormatter(lambda y, _: '{:.2%}'.format(y)))
ax.legend(loc='lower left')
plt.tight_layout()
plt.show()
###Output
_____no_output_____ |
short_notebook/chapter07_short_ver.ipynb | ###Markdown
Library
###Code
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.colors import ListedColormap
from prml.utils.datasets import ClassificationDataGenerator2,RegressionDataGenerator
from prml.sparse_kernel_machines import (
SupportVectorMachineClassifier,
RelevanceVectorMachineRegressor,
RelevanceVectorMachineClassifier
)
def f(x):
return x*x*x - 3*x
def g(x):
return np.sin(x)
cmaps = [[0.122, 0.467, 0.706],"orange","green"]
###Output
_____no_output_____
###Markdown
Support Vector Machine
###Code
gen = ClassificationDataGenerator2(f)
n_data = 200
X_tr,y_tr = gen(encoding="label",x_lower=-3,x_upper=3,y_lower=-3,y_upper=3,n=n_data)
svm = SupportVectorMachineClassifier(C=100.0,kernel="Gaussian",sigma=0.5)
svm.fit(X_tr,y_tr)
print(f"number of support vector {svm.number_of_support_vector()}")
print(f"number of data {n_data}")
cmap = ListedColormap(["red","blue"])
# prepare data
x_min,y_min = X_tr.min(axis = 0)
x_max,y_max = X_tr.max(axis = 0)
x_min,y_min = x_min-0.1,y_min-0.1
x_max,y_max = x_max+0.1,y_max+0.1
x = np.linspace(x_min,x_max,100)
y = np.linspace(y_min,y_max,100)
xs,ys = np.meshgrid(x,y)
# predict
labels = svm.predict(np.array([xs.ravel(),ys.ravel()]).T)
labels = labels.reshape(xs.shape)
# plot
figure,axes = plt.subplots(1,1,figsize=(10,7))
axes.contourf(xs,ys,labels,alpha=0.3,cmap=cmap)
axes.set_xlim(x_min,x_max)
axes.set_ylim(y_min,y_max)
ind_support_vector = svm.index_of_support_vector()
not_support_vector = np.logical_not(np.isin(np.arange(n_data),ind_support_vector))
X_notsv,y_notsv = X_tr[not_support_vector],y_tr[not_support_vector]
X_sv,y_sv = X_tr[ind_support_vector],y_tr[ind_support_vector]
axes.scatter(x=X_notsv[y_notsv == -1,0],y=X_notsv[y_notsv == -1,1],alpha=0.8,c="red",label=-1,s=20)
axes.scatter(x=X_notsv[y_notsv == 1,0],y=X_notsv[y_notsv == 1,1],alpha=0.8,c="blue",label=1,s=20)
axes.scatter(x=X_sv[y_sv == -1,0],y=X_sv[y_sv == -1,1],alpha=0.8,c="red",label=f"support vector {-1}",marker="^",s=60)
axes.scatter(x=X_sv[y_sv == 1,0],y=X_sv[y_sv == 1,1],alpha=0.8,c="blue",label=f"support vector {1}",marker="^",s=60)
axes.set_title("Support Vector Machine")
plt.legend()
plt.show()
###Output
_____no_output_____
###Markdown
Relevance Vector Machine Regressor
###Code
# training
n_data = 100
rvm = RelevanceVectorMachineRegressor(kernel="Gaussian",sigma=2,max_iter=1000)
generator = RegressionDataGenerator(g)
X_tr,y_tr = generator(lower=0,upper=2*np.pi,std=0.2,n=n_data)
rvm.fit(X_tr,y_tr,optimize_param=True)
print(f"number of relevance vector: {rvm.number_of_relevance_vector()}")
print(f"number of daat: {n_data}")
# inference
X = np.linspace(0,2*np.pi,100).reshape(-1,1)
y_pred,y_std = rvm.predict(X,return_std=True)
y_true = g(X)
rmse = np.mean((y_pred - y_true)**2)**0.5
print(f"RMSE : {rmse}")
fig,ax = plt.subplots(1,1,figsize = (10,7))
ax.plot(X,y_pred,label="Predict",color=cmaps[0])
y_pred_upper = y_pred + y_std
y_pred_lower = y_pred - y_std
ax.fill_between(X.ravel(),y_pred_lower.ravel(),y_pred_upper.ravel(),alpha=0.3,color=cmaps[0])
ax.plot(X,y_true,label="Ground Truth",color=cmaps[1])
ax.set_title("Relevance Vector")
ind_relevance_vector = rvm.index_of_relevance_vector()
not_relevance_vector = np.logical_not(np.isin(np.arange(n_data),ind_relevance_vector))
X_notrv,y_notrv = X_tr[not_relevance_vector],y_tr[not_relevance_vector]
X_rv,y_rv = X_tr[ind_relevance_vector],y_tr[ind_relevance_vector]
ax.scatter(x=X_notrv[:,0],y=y_notrv[:,0],alpha=0.8,c=cmaps[2],label="training data",s=20)
ax.scatter(x=X_rv[:,0],y=y_rv[:,0],alpha=0.8,c=cmaps[2],label=f"relevance vector",marker="^",s=80)
plt.legend()
plt.show()
###Output
number of relevance vector: 8
number of daat: 100
RMSE : 0.04091119804863553
###Markdown
Relevance Vector Machine Classifier
###Code
en = ClassificationDataGenerator2(f)
n_data = 50
X_tr,y_tr = gen(encoding="label",x_lower=-3,x_upper=3,y_lower=-3,y_upper=3,n=n_data)
#X_tr,y_tr = gen(encoding="label",x_lower=0,x_upper=7,y_lower=0,y_upper=7,n=n_data)
rvm = RelevanceVectorMachineClassifier(max_iter=100,threshold=1e-8,kernel="Gaussian",sigma=0.5)
rvm.fit(X_tr,y_tr)
print(f"Number of relevance vector: {rvm.number_of_relevance_vector()}")
print(f"Number of data: {n_data}")
cmap = ListedColormap(["red","blue"])
# prepare data
x_min,y_min = X_tr.min(axis = 0)
x_max,y_max = X_tr.max(axis = 0)
x_min,y_min = x_min-0.1,y_min-0.1
x_max,y_max = x_max+0.1,y_max+0.1
x = np.linspace(x_min,x_max,100)
y = np.linspace(y_min,y_max,100)
xs,ys = np.meshgrid(x,y)
# predict
labels = rvm.predict(np.array([xs.ravel(),ys.ravel()]).T)
labels = labels.reshape(xs.shape)
figure,axes = plt.subplots(1,1,figsize=(10,7))
axes.contourf(xs,ys,labels,alpha=0.3,cmap=cmap)
axes.set_xlim(x_min,x_max)
axes.set_ylim(y_min,y_max)
ind_relevance_vector = rvm.index_of_relevance_vector()
not_relevance_vector = np.logical_not(np.isin(np.arange(n_data),ind_relevance_vector))
X_notrv,y_notrv = X_tr[not_relevance_vector],y_tr[not_relevance_vector]
X_rv,y_rv = X_tr[ind_relevance_vector],y_tr[ind_relevance_vector]
axes.scatter(x=X_notrv[y_notrv == 0,0],y=X_notrv[y_notrv == 0,1],alpha=0.8,c="red",label=0,s=20)
axes.scatter(x=X_notrv[y_notrv == 1,0],y=X_notrv[y_notrv == 1,1],alpha=0.8,c="blue",label=1,s=20)
axes.scatter(x=X_rv[y_rv == 0,0],y=X_rv[y_rv == 0,1],alpha=0.8,c="red",label=f"relevance vector {0}",marker="^",s=60)
axes.scatter(x=X_rv[y_rv == 1,0],y=X_rv[y_rv == 1,1],alpha=0.8,c="blue",label=f"relevance vector {1}",marker="^",s=60)
axes.set_title("Relevance Vector Machine")
plt.legend()
plt.show()
###Output
Number of relevance vector: 14
Number of data: 50
|
src/Example-Notebook.ipynb | ###Markdown
Template Repository for Research Papers with Python CodeMain Code to reproduce the results in the paper
###Code
import logging
import matplotlib.pyplot as plt
import seaborn as sns
from sklearn.preprocessing import StandardScaler
from sklearn.model_selection import RandomizedSearchCV
from sklearn.utils.fixes import loguniform
from scipy.stats import uniform
from file_handling import (
load_data, export_results, serialize_model, deserialize_model)
from preprocessing import select_features
from pyrcn.extreme_learning_machine import ELMRegressor
###Output
_____no_output_____
###Markdown
To suppress functionality, you can easily deactivate these options
###Code
plot=False
export=True
serialize=True
###Output
_____no_output_____
###Markdown
At first, we load the training dataset
###Code
training_data = load_data("../data/train.csv")
if plot:
fig, axs = plt.subplots()
sns.scatterplot(
data=training_data, x="GrLivArea", y="SalePrice", ax=axs)
plt.title("Training data")
plt.tight_layout()
###Output
_____no_output_____
###Markdown
Next, we scale the input data to have zero mean and unitary a variance of 1, and transform our training data
###Code
X, y, feature_trf = select_features(
df=training_data, input_features=["GrLivArea"], target="SalePrice")
scaler = StandardScaler().fit(X)
X_train = scaler.transform(X)
y_train = y
###Output
_____no_output_____
###Markdown
In case a pre-trained model is available, we can load this.If not, we train our model. If desired, we serialize the model.
###Code
try:
model = deserialize_model("../results/model.joblib")
except FileNotFoundError:
model = RandomizedSearchCV(
estimator=ELMRegressor(input_activation="relu", random_state=42,
hidden_layer_size=50),
param_distributions={"input_scaling": uniform(loc=0, scale=2),
"bias_scaling": uniform(loc=0, scale=2),
"alpha": loguniform(1e-5, 1e1)},
random_state=42, n_iter=200, refit=True).fit(X, y)
if serialize:
serialize_model(model, "../results/model.joblib")
###Output
_____no_output_____
###Markdown
We can visualize how bad this model performs on the training data.
###Code
if plot:
y_pred = model.predict(X)
fig, axs = plt.subplots()
sns.scatterplot(
data=training_data, x="GrLivArea", y="SalePrice", ax=axs)
plt.title("Training data")
sns.scatterplot(x=training_data["GrLivArea"], y=y_pred, ax=axs)
plt.tight_layout()
###Output
_____no_output_____
###Markdown
Next, we load the test dataset, scale it using the fitted scaler and predict the house prices.
###Code
test_data = load_data("../data/test.csv")
X = feature_trf.transform(test_data)
X_test = scaler.transform(X)
y_pred = model.predict(X_test)
###Output
_____no_output_____
###Markdown
We can visualize how bad this model performs on the test data.
###Code
if plot:
fig, axs = plt.subplots()
sns.scatterplot(x=X, y=y_pred, ax=axs)
plt.ylabel("Predicted SalePrice")
plt.title("Test data")
plt.tight_layout()
###Output
_____no_output_____
###Markdown
Finally, we export our results if desired.
###Code
results = {
"GrLivArea": test_data["GrLivArea"], "PredictedSalePrice": y_pred.ravel()}
if export:
export_results(results, "../results/results.csv")
###Output
_____no_output_____ |
03 Feature Selection/Feature_Selection.ipynb | ###Markdown
Machine Learning Model Building Project: Feature SelectionIn the following notebooks, I will take you through a practical example of each one of the steps in the Machine Learning model building pipeline that I learned throughout my experience and analyzing many kaggle notebooks. There will be a notebook for each one of the Machine Learning Pipeline steps:1. Data Analysis2. Feature Engineering3. Feature Selection4. Model Building**This is the notebook for step 3: Feature Selection**We will use the house price dataset available on [Kaggle.com](https://www.kaggle.com/c/house-prices-advanced-regression-techniques/data). See below for more details.=================================================================================================== Predicting Sale Price of HousesThe aim of the project is to build a machine learning model to predict the sale price of homes based on different explanatory variables describing aspects of residential houses. Why is this important? Predicting house prices is useful to identify fruitful investments, or to determine whether the price advertised for a house is over or underestimated, before making a buying judgment. What is the objective of the machine learning model?We aim to minimise the difference between the real price, and the estimated price by our model. We will evaluate model performance using the mean squared error (mse) and the root squared of the mean squared error (rmse). How do I download the dataset?To download the House Price dataset go this website:https://www.kaggle.com/c/house-prices-advanced-regression-techniques/data==================================================================================================== House Prices dataset: Feature SelectionIn the following cells, we will select a group of variables, the most predictive ones, to build our machine learning models. Why do we need to select variables?1. For production: Fewer variables mean smaller client input requirements (e.g. customers filling out a form on a website or mobile app), and hence less code for error handling. This reduces the chances of bugs.2. For model performance: Fewer variables mean simpler, more interpretable, less over-fitted models**We will select variables using the Lasso regression: Lasso has the property of setting the coefficient of non-informative variables to zero. This way we can identify those variables and remove them from our final models.** Setting the seedIt is important to note that we are engineering variables and pre-processing data with the idea of deploying the model if we find business value in it. Therefore, from now on, for each step that includes some element of randomness, it is extremely important that we **set the seed**. This way, we can obtain reproducibility between our research and our development code.This is perhaps one of the most important lessons that I learned from my mistakes is **Always set the seeds**.Let's go ahead and load the dataset.
###Code
# to handle datasets
import pandas as pd
import numpy as np
# for plotting
import matplotlib.pyplot as plt
%matplotlib inline
# to build the models
from sklearn.linear_model import Lasso
from sklearn.feature_selection import SelectFromModel
# to visualise al the columns in the dataframe
pd.pandas.set_option('display.max_columns', None)
# load dataset
# We load the datasets with the engineered values: we built and saved these datasets in the previous Notebooks.
X_train = pd.read_csv('xtrain.csv')
X_test = pd.read_csv('xtest.csv')
X_train.head()
# capture the target
y_train = X_train['SalePrice']
y_test = X_test['SalePrice']
# drop unnecessary variables from our training and testing sets
X_train.drop(['Id', 'SalePrice'], axis=1, inplace=True)
X_test.drop(['Id', 'SalePrice'], axis=1, inplace=True)
###Output
_____no_output_____
###Markdown
Feature SelectionLet's go ahead and select a subset of the most predictive features. There is an element of randomness in the Lasso regression, so remember to set the seed.
###Code
# here I will do the model fitting and feature selection
# altogether in one line of code
# first, I specify the Lasso Regression model, and I
# select a suitable alpha (equivalent of penalty).
# The bigger the alpha the less features that will be selected.
# Then I use the selectFromModel object from sklearn, which
# will select the features which coefficients are non-zero
sel_ = SelectFromModel(Lasso(alpha=0.005, random_state=0)) # remember to set the seed, the random state in this function
sel_.fit(X_train, y_train)
# this command let's us visualise those features that were kept.
# Kept features have a True indicator
sel_.get_support()
# let's print the number of total and selected features
# this is how we can make a list of the selected features
selected_feat = X_train.columns[(sel_.get_support())]
# let's print some stats
print('total features: {}'.format((X_train.shape[1])))
print('selected features: {}'.format(len(selected_feat)))
print('features with coefficients shrank to zero: {}'.format(
np.sum(sel_.estimator_.coef_ == 0)))
# print the selected features
selected_feat
###Output
_____no_output_____
###Markdown
Identify the selected variables
###Code
# this is an alternative way of identifying the selected features
# based on the non-zero regularisation coefficients:
selected_feats = X_train.columns[(sel_.estimator_.coef_ != 0).ravel().tolist()]
selected_feats
# now we save the selected list of features
pd.Series(selected_feats).to_csv('selected_features.csv', index=False)
###Output
_____no_output_____ |
notebooks/Probabilities_AmeliaTaylor_Mine.ipynb | ###Markdown
Probability Problems A short discussion of \ 1 from the exercies from Peter KomarThe probability of rain on Saturday and Sunday are 50% and 20%, respectively. Any rain will be lightwith 90%, and heavy with 10% probability on Saturday. Any rain on Sunday will be light.Q1: What is the probability of light rain on both days?
###Code
P = 0.5*.9 * .2*1.
print(P)
###Output
0.09
###Markdown
Q2: What is the probability of raining during the weekend?
###Code
P = .5*.8 + .5*.2 + .5*.2
print(P)
###Output
0.6
###Markdown
Inference and probability problems.1. A student is taking a one-hour-time-limit makeup examination. Suppose the probability that the student will finish the exam in less than x hours is x/2, for all 0<= x<= 1. Fiven that the student is still working after .75 hours, what is the conditional probability that the full hour is used?
###Code
# Bayes: P(A|B) = P(B|A)*P(A)/P(B) = P(AB)/P(B) where P(AB) = A intersect B, so both events happen
L_x = event they finish in x hour
P(L_x) = x/2
P(1 | )
0.8
###Output
_____no_output_____
###Markdown
2. An insurance company believes that people can be divided into two classes: those who are accident prone and those who are not. Their statistics show that an accident-prone person will have an accident at some time within a fixed 1-year period with probability 0.4, whereas this probability decreases to 0.2 for a non-accident-prone person. If we assume that 30 percent of the population is accident prone, what is the probability that a new policyholder will have an accident within a year of purchasing a policy? As a follow-up, suppose that a new policyholder has an accident within ayear of purchasing a policy. What is the probability she is accident prone?
###Code
# L_1 = event that accident happen within one year
#P(AP) = .3
#P(NAP) = .7
#P(A|AP) = .4
#P(A|NAP) = .2
#P(A) = P(A|AP)*P(A) + P(A|NAP)*P(NAP)
P = .4*.3+.2*.7
print(P)
# P(AP|A) = P(A|AP)*P(AP)/(P(A|AP)*P(AP) + P(A|NAP)*P(NAP))
P2 = .4*.3/(.4*.3 + .2*.7)
print(P2)
###Output
0.26
0.461538461538
###Markdown
3. There are three types of coins which have different probabilities of landing heads when tossed. * Type A coins are fair, with probability .5 of heads * Type B coins are bent and have probability .6 of heads * Type C coins are bent and have probability .9 of headsSuppose I have a drawer containing 4 coins: 2 of type A, 1 of type B, and 1 of type C. I reach into the drawer and pick a coin at random. Without showing you the coin I flip it once and get heads. What is the probability it is type A? Type B? Type C?
###Code
# PRIOR PROBABILITIES
PA = 2./4
PB = 1./4
PC = 1./4
PhA = .5
PhB = .6
PhC = .9
sumA = PhA*PA + PhB*PB + PhC*PC
PAh = PhA*PA/sumA
PBh = PhB*PB/sumA
PCh = PhC*PC/sumA
print(PAh)
print(PBh)
print(PCh)
###Output
0.4
0.24
0.36
###Markdown
Expected value problems1. There are 2 coins in a bin. When one of them is flipped it lands on heads with probability 0.6, and when the other coin is flipped it lands on heads with probability 0.3. One of these coins is chosen randomly and then flipped. Without knowing which coin is chosen, you can bet any amount up to 10 dollars and then you either win that amount if teh coin comes up heads aor lose it if it comes up tails. Suppose however, that an insider is willing to selly ou, for an amount C, the information as to whihc coin was selected. What is your expected payoff if you buy this information?
###Code
PC1 = 0.5
PC2 = 0.5
PhC1 = .6
PhC2 = .3
X = 20;
EX = PC1*PhC1*X + PC1*(1-PhC1)*-X + PC2*PhC2*X + PC2*(1-PhC2)*-X
print(EX)
print('EX is -X/10, where X is between [0,10]')
EX2 = PC1*PhC1*X + PC1*(1-PhC1)*-X + PC2*PhC2*X + PC2*(1-PhC2)*-X
###Output
-2.0
EX is X/10, where X is between [0,10]
###Markdown
2. At the end of basketball practice, a team’s best free throw shooter (80%) steps to the line. If she makes the free throw, practice is over. If, on the other hand, she misses, the entire team must run a “killer”, the player tries to shoot another free throw, and the process is repeated until she finally makes a free throw. What are the expected number of “killers” the team will run? What assumptions are being made?
###Code
PT = 0.8
PKT = 0.
PKnT = 1.
###Output
_____no_output_____ |
NASA_telomeres/.ipynb_checkpoints/01a NASA Astros Telos Data Extraction-checkpoint.ipynb | ###Markdown
--- The telomere length measurements by telomere FISH are stored in individual excel sheets per timepoint per individual. Thus, we have roughly 200 excel sheets to extract data from. Data will be extracted into a dict from which a dataframe is created and saved as a .csv for later use. --- Extracting telomere length data by FISH for all astronauts into a dict
###Code
# this function pulls individual telomere length data from the excel sheets containing
# ImageJ telometer data. DAPI, missing values, and values greater than
# 3 standard devs from the mean are purged. all telo measurements
# are standardized according to microscope imaged w/, using Cy3 bead values
# to control for microscope intensity differences
dict_astro_individ_telos_dfs = telo_ma.generate_dictionary_for_telomere_length_data(
'../../names cleaned every astro telo excel sheet')
###Output
dso7673_L-60.xlsx telomere data acquisition in progress..
dso7673_R+270.xlsx telomere data acquisition in progress..
dso2381_L-60.xlsx telomere data acquisition in progress..
dso2494_R+7.xlsx telomere data acquisition in progress..
dso2494_L-270.xlsx telomere data acquisition in progress..
dso2381_R+180.xlsx telomere data acquisition in progress..
dso1536_R+60.xlsx telomere data acquisition in progress..
dso2494_L-180.xlsx telomere data acquisition in progress..
dso1536_R+7.xlsx telomere data acquisition in progress..
dso1062_R+7.xlsx telomere data acquisition in progress..
dso2381_R+270.xlsx telomere data acquisition in progress..
dso7673_R+180.xlsx telomere data acquisition in progress..
dso2171_L-180.xlsx telomere data acquisition in progress..
dso1536_FD140.xlsx telomere data acquisition in progress..
dso1536_L-60.xlsx telomere data acquisition in progress..
dso3228_R+180.xlsx telomere data acquisition in progress..
dso4819_L-180.xlsx telomere data acquisition in progress..
dso3228_R+7.xlsx telomere data acquisition in progress..
dso2381_R+60.xlsx telomere data acquisition in progress..
dso1536_L-180.xlsx telomere data acquisition in progress..
dso7673_R+60.xlsx telomere data acquisition in progress..
dso4819_L-270.xlsx telomere data acquisition in progress..
dso1536_L-270.xlsx telomere data acquisition in progress..
dso5163_R+7.xlsx telomere data acquisition in progress..
dso3228_R+270.xlsx telomere data acquisition in progress..
dso3228_L-60.xlsx telomere data acquisition in progress..
dso2479_L-60.xlsx telomere data acquisition in progress..
dso3228_R+60.xlsx telomere data acquisition in progress..
dso1261_L-180.xlsx telomere data acquisition in progress..
dso2479_R+270.xlsx telomere data acquisition in progress..
dso1536_FD90.xlsx telomere data acquisition in progress..
dso1062_L-180.xlsx telomere data acquisition in progress..
dso2479_R+180.xlsx telomere data acquisition in progress..
dso2479_R+60.xlsx telomere data acquisition in progress..
dso1062_L-270.xlsx telomere data acquisition in progress..
dso5163_R+180.xlsx telomere data acquisition in progress..
dso4819_R+7.xlsx telomere data acquisition in progress..
dso1261_L-270.xlsx telomere data acquisition in progress..
dso1062_L-60.xlsx telomere data acquisition in progress..
dso2381_L-270.xlsx telomere data acquisition in progress..
dso2494_R+180.xlsx telomere data acquisition in progress..
dso4819_L-60.xlsx telomere data acquisition in progress..
dso1261_L-60.xlsx telomere data acquisition in progress..
dso7673_L-180.xlsx telomere data acquisition in progress..
dso7673_L-270.xlsx telomere data acquisition in progress..
dso2494_R+60.xlsx telomere data acquisition in progress..
dso2171_L-60.xlsx telomere data acquisition in progress..
dso5163_R+60.xlsx telomere data acquisition in progress..
dso2171_FD260.xlsx telomere data acquisition in progress..
dso2381_L-180.xlsx telomere data acquisition in progress..
dso2494_R+270.xlsx telomere data acquisition in progress..
dso1536_R+270.xlsx telomere data acquisition in progress..
dso4819_R+270.xlsx telomere data acquisition in progress..
dso1261_R+60.xlsx telomere data acquisition in progress..
dso4819_R+60.xlsx telomere data acquisition in progress..
dso3228_L-270.xlsx telomere data acquisition in progress..
dso2479_R+7.xlsx telomere data acquisition in progress..
dso1062_R+60.xlsx telomere data acquisition in progress..
dso3228_L-180.xlsx telomere data acquisition in progress..
dso5163_L-60.xlsx telomere data acquisition in progress..
dso2171_R+180.xlsx telomere data acquisition in progress..
dso2494_L-60.xlsx telomere data acquisition in progress..
dso1536_R+180.xlsx telomere data acquisition in progress..
dso4819_R+180.xlsx telomere data acquisition in progress..
DSO2171_R+105.xlsx telomere data acquisition in progress..
dso2171_FD45.xlsx telomere data acquisition in progress..
dso1062_R+270.xlsx telomere data acquisition in progress..
dso2479_L-180.xlsx telomere data acquisition in progress..
dso5163_FD140.xlsx telomere data acquisition in progress..
dso1261_R+270.xlsx telomere data acquisition in progress..
dso1261_R+7.xlsx telomere data acquisition in progress..
dso5163_L-180.xlsx telomere data acquisition in progress..
dso2381_R+7.xlsx telomere data acquisition in progress..
dso2171_R+5.xlsx telomere data acquisition in progress..
dso5163_L-270.xlsx telomere data acquisition in progress..
dso1261_R+180.xlsx telomere data acquisition in progress..
dso1062_R+180.xlsx telomere data acquisition in progress..
dso2479_L-270.xlsx telomere data acquisition in progress..
dso5163_FD90.xlsx telomere data acquisition in progress..
Done collecting all astronaut telomere length excel files
###Markdown
Making a dataframe from the dict
###Code
# takes data from above dict, standardizes # of telomeres according to theoretical max
# for these samples (184 per metaphase, 30 metaphases each sample) using
# random sampling from data per sample
# makes dataframe
astro_df = telo_ma.make_astronaut_dataframe(dict_astro_individ_telos_dfs)
astro_df.head(2)
###Output
_____no_output_____
###Markdown
Saving all astros telo dataframe to csv for later retrieval
###Code
copy_astro_df = astro_df
copy_astro_df['telo data'] = copy_astro_df['telo data'].apply(lambda row: row.tolist())
copy_astro_df.to_csv('../excel data/All_astronauts_telomere_length_dataframe.csv', index = False)
###Output
_____no_output_____
###Markdown
Extracting telomere length data by FISH for all astronaut controls into a dict
###Code
dict_ctrl_individ_telos_dfs = telo_ma.grab_control_values_generate_dictionary('../../control files')
###Output
tsf3907 mphase TeloFISH ___ L-180.xlsx IT WORKS PEGGY!!! <3
tsf2580 mphase TeloFISH L-180.xlsx IT WORKS PEGGY!!! <3
tsf3609 mphase TeloFISH R+7.xlsx IT WORKS PEGGY!!! <3
tsf3609 mphase TeloFISH L-270.xlsx IT WORKS PEGGY!!! <3
tsf4127 mphase TeloFISH R+7.xlsx IT WORKS PEGGY!!! <3
tsf1264 mphase TeloFISH R+180.xlsx IT WORKS PEGGY!!! <3
tsf1264 mphase TeloFISH R+270.xlsx IT WORKS PEGGY!!! <3
tsf3609 mphase TeloFISH L-180.xlsx IT WORKS PEGGY!!! <3
tsf3907 mphase TeloFISH ___ L-270.xlsx IT WORKS PEGGY!!! <3
tsf2580 mphase TeloFISH L-270.xlsx IT WORKS PEGGY!!! <3
tsf1826 mphase TeloFISH ___ R+7.xlsx IT WORKS PEGGY!!! <3
tsf0397 mphase TeloFISH R+180.xlsx IT WORKS PEGGY!!! <3
TSF0646_R+7.xlsx IT WORKS PEGGY!!! <3
TSF0912_FD45.xlsx IT WORKS PEGGY!!! <3
tsf0397 mphase TeloFISH R+270.xlsx IT WORKS PEGGY!!! <3
TSF0912_R+270.xlsx IT WORKS PEGGY!!! <3
tsf1264 mphase TeloFISH R+60.xlsx IT WORKS PEGGY!!! <3
tsf4127 mphase TeloFISH R+180.xlsx IT WORKS PEGGY!!! <3
tsf4127 mphase TeloFISH R+270.xlsx IT WORKS PEGGY!!! <3
TSF0912_R+7.xlsx IT WORKS PEGGY!!! <3
tsf2377 mphase TeloFISH R+7.xlsx IT WORKS PEGGY!!! <3
tsf3907 mphase TeloFISH ___ L-60.xlsx IT WORKS PEGGY!!! <3
TSF0646_R+180.xlsx IT WORKS PEGGY!!! <3
tsf1826 mphase TeloFISH ___ L-270.xlsx IT WORKS PEGGY!!! <3
tsf2377 mphase TeloFISH L-180.xlsx IT WORKS PEGGY!!! <3
tsf2377 mphase TeloFISH L-270.xlsx IT WORKS PEGGY!!! <3
TSF0912_FD260.xlsx IT WORKS PEGGY!!! <3
tsf3907 mphase TeloFISH ___ R+60.xlsx IT WORKS PEGGY!!! <3
TSF0646_R+270.xlsx IT WORKS PEGGY!!! <3
tsf1826 mphase TeloFISH ___ L-180.xlsx IT WORKS PEGGY!!! <3
tsf3609 mphase TeloFISH R+180.xlsx IT WORKS PEGGY!!! <3
tsf1264 mphase TeloFISH R+7.xlsx IT WORKS PEGGY!!! <3
tsf1264 mphase TeloFISH L-270.xlsx IT WORKS PEGGY!!! <3
tsf0100 R+60.xlsx IT WORKS PEGGY!!! <3
tsf2580 mphase TeloFISH R+60.xlsx IT WORKS PEGGY!!! <3
tsf2580 mphase TeloFISH R+270.xlsx IT WORKS PEGGY!!! <3
tsf2580 mphase TeloFISH R+7.xlsx IT WORKS PEGGY!!! <3
tsf2580 mphase TeloFISH R+180.xlsx IT WORKS PEGGY!!! <3
tsf1264 mphase TeloFISH L-180.xlsx IT WORKS PEGGY!!! <3
tsf1826 mphase TeloFISH ___ L-60.xlsx IT WORKS PEGGY!!! <3
tsf3609 mphase TeloFISH R+270.xlsx IT WORKS PEGGY!!! <3
tsf0100 L-270.xlsx IT WORKS PEGGY!!! <3
tsf0397 mphase TeloFISH L-270.xlsx IT WORKS PEGGY!!! <3
tsf2580 mphase TeloFISH L-60.xlsx IT WORKS PEGGY!!! <3
tsf0100 L-60.xlsx IT WORKS PEGGY!!! <3
tsf1826 mphase TeloFISH ___ R+60.xlsx IT WORKS PEGGY!!! <3
tsf0397 mphase TeloFISH L-180.xlsx IT WORKS PEGGY!!! <3
tsf3907 mphase TeloFISH ___ R+7.xlsx IT WORKS PEGGY!!! <3
TSF0646_L-60.xlsx IT WORKS PEGGY!!! <3
tsf0100 L-180.xlsx IT WORKS PEGGY!!! <3
tsf0397 mphase TeloFISH L-60.xlsx IT WORKS PEGGY!!! <3
tsf4127 mphase TeloFISH R+60.xlsx IT WORKS PEGGY!!! <3
tsf4127 mphase TeloFISH L-270.xlsx IT WORKS PEGGY!!! <3
TSF0912_L-180.xlsx IT WORKS PEGGY!!! <3
tsf2377 mphase TeloFISH L-60.xlsx IT WORKS PEGGY!!! <3
tsf3609 mphase TeloFISH L-60.xlsx IT WORKS PEGGY!!! <3
tsf4127 mphase TeloFISH L-180.xlsx IT WORKS PEGGY!!! <3
tsf2377 mphase TeloFISH R+270.xlsx IT WORKS PEGGY!!! <3
tsf4127 mphase TeloFISH L-60.xlsx IT WORKS PEGGY!!! <3
tsf0397 mphase TeloFISH R+60.xlsx IT WORKS PEGGY!!! <3
tsf1826 mphase TeloFISH ___ R+180.xlsx IT WORKS PEGGY!!! <3
tsf1826 mphase TeloFISH ___ R+270.xlsx IT WORKS PEGGY!!! <3
tsf3609 mphase TeloFISH R+60.xlsx IT WORKS PEGGY!!! <3
TSF0646_L-180.xlsx IT WORKS PEGGY!!! <3
TSF0646_FD45.xlsx IT WORKS PEGGY!!! <3
tsf2377 mphase TeloFISH R+180.xlsx IT WORKS PEGGY!!! <3
tsf2377 mphase TeloFISH R+60.xlsx IT WORKS PEGGY!!! <3
data collection complete
###Markdown
Making dataframe from dict
###Code
# same as above
control_df = telo_ma.make_control_dataframe(dict_ctrl_individ_telos_dfs)
control_df['flight status'] = 'Controls'
control_df.head(2)
###Output
_____no_output_____
###Markdown
Saving all astro controls telo data for later retrieval
###Code
copy_control_df = control_df
copy_control_df['telo data'] = copy_control_df['telo data'].apply(lambda row: row.tolist())
copy_control_df.to_csv('../excel data/All_astro_controls_telomere_length_dataframe.csv', index=False)
###Output
_____no_output_____ |
PythonDataScienceHandbook-master/notebooks/04.11-Settings-and-Stylesheets.ipynb | ###Markdown
*This notebook contains an excerpt from the [Python Data Science Handbook](http://shop.oreilly.com/product/0636920034919.do) by Jake VanderPlas; the content is available [on GitHub](https://github.com/jakevdp/PythonDataScienceHandbook).**The text is released under the [CC-BY-NC-ND license](https://creativecommons.org/licenses/by-nc-nd/3.0/us/legalcode), and code is released under the [MIT license](https://opensource.org/licenses/MIT). If you find this content useful, please consider supporting the work by [buying the book](http://shop.oreilly.com/product/0636920034919.do)!* Customizing Matplotlib: Configurations and Stylesheets Matplotlib's default plot settings are often the subject of complaint among its users.While much is slated to change in the 2.0 Matplotlib release in late 2016, the ability to customize default settings helps bring the package inline with your own aesthetic preferences.Here we'll walk through some of Matplotlib's runtime configuration (rc) options, and take a look at the newer *stylesheets* feature, which contains some nice sets of default configurations. Plot Customization by HandThrough this chapter, we've seen how it is possible to tweak individual plot settings to end up with something that looks a little bit nicer than the default.It's possible to do these customizations for each individual plot.For example, here is a fairly drab default histogram:
###Code
import matplotlib.pyplot as plt
plt.style.use('classic')
import numpy as np
%matplotlib inline
x = np.random.randn(1000)
plt.hist(x);
###Output
_____no_output_____
###Markdown
We can adjust this by hand to make it a much more visually pleasing plot:
###Code
# use a gray background
ax = plt.axes(axisbg='#E6E6E6')
ax.set_axisbelow(True)
# draw solid white grid lines
plt.grid(color='w', linestyle='solid')
# hide axis spines
for spine in ax.spines.values():
spine.set_visible(False)
# hide top and right ticks
ax.xaxis.tick_bottom()
ax.yaxis.tick_left()
# lighten ticks and labels
ax.tick_params(colors='gray', direction='out')
for tick in ax.get_xticklabels():
tick.set_color('gray')
for tick in ax.get_yticklabels():
tick.set_color('gray')
# control face and edge color of histogram
ax.hist(x, edgecolor='#E6E6E6', color='#EE6666');
###Output
_____no_output_____
###Markdown
This looks better, and you may recognize the look as inspired by the look of the R language's ggplot visualization package.But this took a whole lot of effort!We definitely do not want to have to do all that tweaking each time we create a plot.Fortunately, there is a way to adjust these defaults once in a way that will work for all plots. Changing the Defaults: ``rcParams``Each time Matplotlib loads, it defines a runtime configuration (rc) containing the default styles for every plot element you create.This configuration can be adjusted at any time using the ``plt.rc`` convenience routine.Let's see what it looks like to modify the rc parameters so that our default plot will look similar to what we did before.We'll start by saving a copy of the current ``rcParams`` dictionary, so we can easily reset these changes in the current session:
###Code
IPython_default = plt.rcParams.copy()
###Output
_____no_output_____
###Markdown
Now we can use the ``plt.rc`` function to change some of these settings:
###Code
from matplotlib import cycler
colors = cycler('color',
['#EE6666', '#3388BB', '#9988DD',
'#EECC55', '#88BB44', '#FFBBBB'])
plt.rc('axes', facecolor='#E6E6E6', edgecolor='none',
axisbelow=True, grid=True, prop_cycle=colors)
plt.rc('grid', color='w', linestyle='solid')
plt.rc('xtick', direction='out', color='gray')
plt.rc('ytick', direction='out', color='gray')
plt.rc('patch', edgecolor='#E6E6E6')
plt.rc('lines', linewidth=2)
###Output
_____no_output_____
###Markdown
With these settings defined, we can now create a plot and see our settings in action:
###Code
plt.hist(x);
###Output
_____no_output_____
###Markdown
Let's see what simple line plots look like with these rc parameters:
###Code
for i in range(4):
plt.plot(np.random.rand(10))
###Output
_____no_output_____
###Markdown
I find this much more aesthetically pleasing than the default styling.If you disagree with my aesthetic sense, the good news is that you can adjust the rc parameters to suit your own tastes!These settings can be saved in a *.matplotlibrc* file, which you can read about in the [Matplotlib documentation](http://Matplotlib.org/users/customizing.html).That said, I prefer to customize Matplotlib using its stylesheets instead. StylesheetsThe version 1.4 release of Matplotlib in August 2014 added a very convenient ``style`` module, which includes a number of new default stylesheets, as well as the ability to create and package your own styles. These stylesheets are formatted similarly to the *.matplotlibrc* files mentioned earlier, but must be named with a *.mplstyle* extension.Even if you don't create your own style, the stylesheets included by default are extremely useful.The available styles are listed in ``plt.style.available``—here I'll list only the first five for brevity:
###Code
plt.style.available[:5]
###Output
_____no_output_____
###Markdown
The basic way to switch to a stylesheet is to call``` pythonplt.style.use('stylename')```But keep in mind that this will change the style for the rest of the session!Alternatively, you can use the style context manager, which sets a style temporarily:``` pythonwith plt.style.context('stylename'): make_a_plot()``` Let's create a function that will make two basic types of plot:
###Code
def hist_and_lines():
np.random.seed(0)
fig, ax = plt.subplots(1, 2, figsize=(11, 4))
ax[0].hist(np.random.randn(1000))
for i in range(3):
ax[1].plot(np.random.rand(10))
ax[1].legend(['a', 'b', 'c'], loc='lower left')
###Output
_____no_output_____
###Markdown
We'll use this to explore how these plots look using the various built-in styles. Default styleThe default style is what we've been seeing so far throughout the book; we'll start with that.First, let's reset our runtime configuration to the notebook default:
###Code
# reset rcParams
plt.rcParams.update(IPython_default);
###Output
_____no_output_____
###Markdown
Now let's see how it looks:
###Code
hist_and_lines()
###Output
_____no_output_____
###Markdown
FiveThiryEight styleThe ``fivethirtyeight`` style mimics the graphics found on the popular [FiveThirtyEight website](https://fivethirtyeight.com).As you can see here, it is typified by bold colors, thick lines, and transparent axes:
###Code
with plt.style.context('fivethirtyeight'):
hist_and_lines()
###Output
_____no_output_____
###Markdown
ggplotThe ``ggplot`` package in the R language is a very popular visualization tool.Matplotlib's ``ggplot`` style mimics the default styles from that package:
###Code
with plt.style.context('ggplot'):
hist_and_lines()
###Output
_____no_output_____
###Markdown
*Bayesian Methods for Hackers( styleThere is a very nice short online book called [*Probabilistic Programming and Bayesian Methods for Hackers*](http://camdavidsonpilon.github.io/Probabilistic-Programming-and-Bayesian-Methods-for-Hackers/); it features figures created with Matplotlib, and uses a nice set of rc parameters to create a consistent and visually-appealing style throughout the book.This style is reproduced in the ``bmh`` stylesheet:
###Code
with plt.style.context('bmh'):
hist_and_lines()
###Output
_____no_output_____
###Markdown
Dark backgroundFor figures used within presentations, it is often useful to have a dark rather than light background.The ``dark_background`` style provides this:
###Code
with plt.style.context('dark_background'):
hist_and_lines()
###Output
_____no_output_____
###Markdown
GrayscaleSometimes you might find yourself preparing figures for a print publication that does not accept color figures.For this, the ``grayscale`` style, shown here, can be very useful:
###Code
with plt.style.context('grayscale'):
hist_and_lines()
###Output
_____no_output_____
###Markdown
Seaborn styleMatplotlib also has stylesheets inspired by the Seaborn library (discussed more fully in [Visualization With Seaborn](04.14-Visualization-With-Seaborn.ipynb)).As we will see, these styles are loaded automatically when Seaborn is imported into a notebook.I've found these settings to be very nice, and tend to use them as defaults in my own data exploration.
###Code
import seaborn
hist_and_lines()
###Output
_____no_output_____ |
examples/networks/howtos/map_to_regions.ipynb | ###Markdown
map_to_regions maps Import packages
###Code
import numpy as np
import porespy as ps
import openpnm as op
import matplotlib.pyplot as plt
ws = op.Workspace()
ws.settings['loglevel'] = 50
np.random.seed(10)
ps.visualization.set_mpl_style()
###Output
_____no_output_____
###Markdown
Create image and extract network
###Code
im = ps.generators.blobs(shape=[400, 400], porosity=0.6)
snow_output = ps.networks.snow2(im, boundary_width=10)
###Output
_____no_output_____
###Markdown
Plot the pore network:
###Code
pn, geo = op.io.PoreSpy.import_data(snow_output.network)
fig = plt.figure(figsize=[4, 4])
fig = op.topotools.plot_connections(pn, c='w', linewidth=2, ax=fig)
fig = op.topotools.plot_coordinates(pn, c='w', s=100, ax=fig)
plt.imshow(snow_output.regions.T, origin='lower')
plt.axis('off');
###Output
_____no_output_____
###Markdown
Now assign some values to the network:
###Code
pn['pore.values'] = np.random.rand(pn.Np)
###Output
_____no_output_____
###Markdown
And now assign these values to the image regions:
###Code
reg = ps.networks.map_to_regions(regions=snow_output.regions, values=pn['pore.values'])
plt.imshow(reg)
###Output
_____no_output_____ |
.ipynb_checkpoints/Images_and_Color_Theory-checkpoint.ipynb | ###Markdown
Image HandlingThe introduction of cell phones has made digital images a ubiqioutous object in everyone's life. Though some may long for the days of developed film, there are many benefits to switching to digital image processing. The goal of this notebook is to familiarize you with a hallmark of digital images: separating image color into separate digital channels.Reliance on different colors to convey information is a standard practice in science. By understanding how colors are stored in an image and how we can manipulate the visualization of those colors, we will be able to simulate different spectroscopic practices and eventually combine these with a home-built Raspberry Pi instrument.
###Code
# Imports and settings to make the rest of the notebook work. Don't worry about changing these.
%matplotlib inline
%load_ext autoreload
%autoreload 2
import numpy as np
import matplotlib.pyplot as plt
import skimage.io as io
from skimage.color import rgb2gray
from imageProc import *
###Output
_____no_output_____
###Markdown
Let's consider a familiar photo: the Astro's Logo!  The Astro's logo is built on the colors orange, blue, and white. However, that is not how our computers store that information. Two different color mapping strategies exist for rendering colors: RGB (red, green, blue) and CMYK (Cyan, Magenta, Yellow, and Black).
###Code
# Feel free to substitute any image you are interesed in using. I offere the astros logo below simply as an example
image_url = "astros_logo.png"
my_im_proc = ImageProcessor(image_url)
# Generate a figure and axes to graph the different versions of the Astro's Logo
fig, axs = plt.subplots(nrows=1, ncols=3, figsize=(15,5))
# Iterate through all three color channels: Red, Green, and Blue
channels = my_im_proc.splitColors(style = 'RGB')
for c, ax in zip(range(3), axs):
ax.imshow(channels[c])
ax.set_axis_off()
fig, axs = plt.subplots(nrows=1, ncols=3, figsize=(15,5))
channels = my_im_proc.splitColors(style = 'CMYK')
for c, ax in zip(range(3), axs):
ax.imshow(channels[c])
ax.set_axis_off()
###Output
_____no_output_____
###Markdown
Manipulation of color channels is the primary pathway to building the image filters that have made services like instagram so popular. For a quick tutorial on how to make your own color [filter](https://www.practicepython.org/blog/2016/12/20/instagram-filters-python.html)! Breaking pictures down into their subsequent color levels can help highlight details that are otherwise lost in the agglomeration of all colors. Our goal in science is often to isolate that desired bit of information (the "signal") from the rest of the photo (the "noise"). Image Analyses made possible via Color Channel assessment.Digital images are simply matrices of numbers that are translated into colored pixel maps. Because of this simplicity, there are many types of programmatic alterations that we can make to an image. These types of image manipulations are commonplace in both science and other professions. Using computers, we can:- denoise (clear-up) a messy image - detection of edges in images - hide one image inside of another  Cleaning up a Messy ImageMessy images are a common problem in both scientific and consumer communities. Many different denoising algorithms exist, each with a variation on how lost information is interpolated and recovered. Here, we are going to offer up a brief description of the process and offer an opportunity to denoise an image of your own.
###Code
# If you want to use your own image, feel free to substitute your own file path below
img_file_path = './houston_skyline_2.jpg'
# Image processor will be our primary way of handling images.
my_img_proc = ImageProcessor(img_file_path)
# We are going to add some "noise" to our image first.
noisy = my_img_proc.makeNoisyImage()
plt.imshow(noisy)
plt.axis('off')
###Output
_____no_output_____
###Markdown
Now that we have introduced some level of noise to the image, let's talk about how we can fill in this missing informaiton and clean up the image.There are many different denoising protocols that can be used. Today, we are going to talk about using the Total Variation filter. We use this filter as it is excellent at removing overall noise while maintaining the original edges of the image. It does this by reducing the variance between pixel values. You can find out the nitty-gritty details of this method [here](https://en.wikipedia.org/wiki/Total_variation_denoising).
###Code
denoised_image = my_img_proc.imageDenoise()
fig, axs = plt.subplots(nrows=1, ncols=3, figsize=(21,7))
axs[0].imshow(my_img_proc.returnImage())
axs[1].imshow(noisy)
axs[2].imshow(denoised_image)
###Output
_____no_output_____
###Markdown
In denoising the image, we have succesfully removed much of the random fuzz that we introduced into the image. However, what we have gained in clarity we have lost in vibrance and sharpness. The color depth has disappeared but we now have clean lines for all of the buildings. Edge DetectionSometimes we want to isolate separate continuous regions in an image, like the edges of a lake, the outline of a canyon, or the veins in someone's arms. To do this, we dip again into our color information. Using this digital information, we can isolate the boundaries in the image and highlight where changes are happening most rapidly.We will look at two different edge detection types: [Canny](https://towardsdatascience.com/canny-edge-detection-step-by-step-in-python-computer-vision-b49c3a2d8123) and [Sobel](https://en.wikipedia.org/wiki/Sobel_operator)
###Code
# Enter you custom image under im_url
im_url = 'houston_skyline_2.jpg'
sobel_im = my_img_proc.edgeDetection(im_url = im_url, e_type = 'Sobel')
canny_im = my_img_proc.edgeDetection(im_url = im_url, e_type = 'Canny')
fig, axs = plt.subplots(nrows=1, ncols=2, sharey=True, figsize=(18,9))
axs[0].imshow(canny_im)
axs[0].axis("off")
axs[0].set_title('Canny')
axs[1].imshow(sobel_im)
axs[1].axis("off")
axs[1].set_title('Sobel')
###Output
_____no_output_____
###Markdown
Different edge measures yield different results. Exploratory data analysis is key to picking out what is the best methodology for isolating the key features desired. Steganography - The art of hiding an image in another image.One interesting aspect of digital images is to hide one image in the slight color variations of another. The process, well detailed on [towardsDataScience](https://towardsdatascience.com/steganography-hiding-an-image-inside-another-77ca66b2acb1), involves storing the most significant color variations from one image in the least significant information of another.
###Code
# First we want to declare our host image. This is the image that will be visible after concealment.
host_image_url = './houston_skyline_2.jpg'
# This is the image that we will hide inside of the host image. Important note: Both images must have the same size.
hidden_image_url = './ny_skyline_2.jpg'
my_im_proc = ImageProcessor(host_image_url)
# This method merges our images
merged_image = my_im_proc.hideMyImage(image_url=hidden_image_url, save = False)
# This method retrieves the hidden image out of the host.
unmerged_image = my_im_proc.revealMyImage(image_url='./merged.png', save = False)
fig, axs = plt.subplots(nrows=2, ncols=2, figsize=(18,18))
axs[0,0].imshow(plt.imread(host_image_url))
axs[0,0].set_title('Host Image: Houston')
axs[0,0].axis('off')
axs[0,1].imshow(plt.imread(hidden_image_url))
axs[0,1].set_title('Hidden Image: NYC')
axs[0,1].axis('off')
axs[1,0].imshow(merged_image)
axs[1,0].set_title('Merged Skylines')
axs[1,0].axis('off')
axs[1,1].imshow(unmerged_image)
axs[1,1].set_title('NYC Retrieved')
axs[1,1].axis('off')
plt.tight_layout()
###Output
_____no_output_____ |
1.Data-exploration/Profiles_level4/cell_painting/11.cellpainting_well_matched_null_p_values.ipynb | ###Markdown
Generate a null distribution per well
###Code
import os
import sys
import pickle
import random
import pathlib
import numpy as np
import pandas as pd
from scipy import stats
from statistics import median
from collections import defaultdict
from pycytominer import feature_select
sys.path.append("..")
from null_util import get_null_dist_median_scores, get_null_distribution_replicates
np.random.seed(42)
###Output
_____no_output_____
###Markdown
Define constants
###Code
random_permutation_count = 1000
num_unique_sample_cutoff = 20
metadata_cols_to_drop = [
'Metadata_broad_sample',
'Metadata_pert_id',
'Metadata_dose_recode',
'Metadata_Plate',
'Metadata_Well',
'Metadata_broad_id',
'Metadata_moa',
'broad_id',
'pert_iname',
'moa'
]
cp_level4_path = "cellpainting_lvl4_cpd_replicate_datasets"
cp_file_indicator = ""
output_file = pathlib.Path(
"results", f"well_controlled_percent_replicating_non_parametric_p_values{cp_file_indicator}.tsv"
)
###Output
_____no_output_____
###Markdown
Load data
###Code
# Load common compounds
common_file = pathlib.Path(
"..", "..", "..", "6.paper_figures", "data", "significant_compounds_by_threshold_both_assays.tsv.gz"
)
common_df = pd.read_csv(common_file, sep="\t")
common_compounds = common_df.compound.unique()
print(len(common_compounds))
# Load level 4 replicate profiles
df_level4 = pd.read_csv(
os.path.join(cp_level4_path, f'cp_level4_cpd_replicates{cp_file_indicator}.csv.gz'),
compression='gzip',low_memory = False
)
print(df_level4.shape)
df_level4.head(2)
# Get treatment replicate counts per well
cardinality_df = (
df_level4
.groupby(["pert_iname", "Metadata_Well", "Metadata_dose_recode"])
["Metadata_broad_sample"]
.count()
.reset_index()
.rename(columns={"Metadata_broad_sample": "no_of_replicates"})
)
print(cardinality_df.shape)
cardinality_df.head()
# Load existing median pairwise correlations
df_cpd_med_scores = pd.read_csv(os.path.join(cp_level4_path, f'cpd_replicate_median_scores{cp_file_indicator}.csv'))
df_cpd_med_scores = df_cpd_med_scores.set_index('cpd').rename_axis(None, axis=0).copy()
# Subset to common compound measurements
df_cpd_med_scores = (
df_cpd_med_scores
.loc[df_cpd_med_scores.index.isin(common_compounds), :]
.rename(columns={"cpd_size": "no_of_replicates"})
)
print(df_cpd_med_scores.shape)
df_cpd_med_scores.head()
# Transform median scores to map compound to well
df_cpd_med_scores_melt = df_cpd_med_scores.reset_index().rename(columns={"index": "compound"})
df_cpd_med_scores_melt = (
df_cpd_med_scores_melt
.melt(
id_vars=["compound", "no_of_replicates"],
var_name="dose",
value_name="median_score"
)
).drop("no_of_replicates", axis="columns")
# Merge with important metadata
df_cpd_med_scores_melt = (
df_cpd_med_scores_melt
.assign(
dose_recode=df_cpd_med_scores_melt.dose.str[-1].astype(int)
)
.merge(
df_level4.loc[:, [
"Metadata_Well",
"pert_iname",
"Metadata_dose_recode"
]],
left_on=["compound", "dose_recode"],
right_on=["pert_iname", "Metadata_dose_recode"],
how="left"
)
.merge(
cardinality_df,
on=["pert_iname", "Metadata_Well", "Metadata_dose_recode"],
how="left"
)
.drop_duplicates()
.reset_index(drop=True)
)
print(df_cpd_med_scores_melt.shape)
df_cpd_med_scores_melt.head(2)
###Output
(7572, 8)
###Markdown
Acquire replicate dictionary
###Code
cpd_replicate_info = {}
for well in df_level4.Metadata_Well.unique():
cpd_replicate_info[well] = {}
df_level4_subset = df_level4.query("Metadata_Well == @well")
for cpd in df_level4_subset.pert_iname.unique():
df_level4_cpd_subset = df_level4_subset.query("pert_iname == @cpd")
cpd_replicate_info[well][cpd] = df_level4_cpd_subset.replicate_name.tolist()
###Output
_____no_output_____
###Markdown
Define sampling constraints- Must be same well- Must not include any matching compounds
###Code
# How many unique perturbations per well
(
df_level4
.groupby(["Metadata_Well", "pert_iname"])["Metadata_broad_sample"]
.nunique()
.reset_index()
.Metadata_Well
.value_counts()
.hist()
)
###Output
_____no_output_____
###Markdown
Process null distribution per well
###Code
full_well_null_distribution_of_scores = {}
did_not_pass_info = {}
for well in df_level4.Metadata_Well.unique():
# Print for progress
print(f"Now processing well: {well}")
# Subset the profiles to only one specific well
level4_well_subset_df = df_level4.query("Metadata_Well == @well")
# Make sure there are enough different samples
num_unique_samples = level4_well_subset_df.pert_iname.nunique()
if num_unique_samples <= num_unique_sample_cutoff:
did_not_pass_info[well] = {"num_unique_samples": num_unique_samples}
continue
# Define the replicate cardinalities
replicate_cardinalities = level4_well_subset_df.pert_iname.value_counts().unique()
# Remove replicate cardinality of 1
replicate_cardinalities = np.array([x for x in replicate_cardinalities if x >=2])
# Define a null distribution
null_distrib_replicates = get_null_distribution_replicates(
well_df=level4_well_subset_df,
cardinalities=replicate_cardinalities,
rand_num=random_permutation_count
)
# Get null distribution median scores
median_scores_per_well = get_null_dist_median_scores(
well_df=level4_well_subset_df,
null_distribution=null_distrib_replicates,
metadata_cols_to_drop=metadata_cols_to_drop
)
# Save null distribution per well
full_well_null_distribution_of_scores[well] = median_scores_per_well
###Output
Now processing well: A01
Now processing well: A02
Now processing well: A03
Now processing well: A04
Now processing well: A05
Now processing well: A06
Now processing well: B01
Now processing well: B02
Now processing well: B03
Now processing well: B04
Now processing well: B05
Now processing well: B06
Now processing well: F07
Now processing well: F08
Now processing well: F09
Now processing well: F10
Now processing well: F11
Now processing well: F12
Now processing well: J13
Now processing well: J14
Now processing well: J15
Now processing well: J16
Now processing well: J17
Now processing well: J18
Now processing well: A07
Now processing well: A08
Now processing well: A09
Now processing well: A10
Now processing well: A11
Now processing well: A12
Now processing well: A13
Now processing well: A14
Now processing well: A15
Now processing well: A16
Now processing well: A17
Now processing well: A18
Now processing well: A19
Now processing well: A20
Now processing well: A21
Now processing well: A22
Now processing well: A23
Now processing well: A24
Now processing well: B07
Now processing well: B08
Now processing well: B09
Now processing well: B10
Now processing well: B11
Now processing well: B12
Now processing well: B13
Now processing well: B14
Now processing well: B15
Now processing well: B16
Now processing well: B17
Now processing well: B18
Now processing well: B19
Now processing well: B20
Now processing well: B21
Now processing well: B22
Now processing well: B23
Now processing well: B24
Now processing well: C01
Now processing well: C02
Now processing well: C03
Now processing well: C04
Now processing well: C05
Now processing well: C06
Now processing well: C07
Now processing well: C08
Now processing well: C09
Now processing well: C10
Now processing well: C11
Now processing well: C12
Now processing well: C13
Now processing well: C14
Now processing well: C15
Now processing well: C16
Now processing well: C17
Now processing well: C18
Now processing well: C19
Now processing well: C20
Now processing well: C21
Now processing well: C22
Now processing well: C23
Now processing well: C24
Now processing well: G13
Now processing well: G14
Now processing well: G15
Now processing well: G16
Now processing well: G17
Now processing well: G18
Now processing well: D01
Now processing well: D02
Now processing well: D03
Now processing well: D04
Now processing well: D05
Now processing well: D06
Now processing well: D07
Now processing well: D08
Now processing well: D09
Now processing well: D10
Now processing well: D11
Now processing well: D12
Now processing well: D13
Now processing well: D14
Now processing well: D15
Now processing well: D16
Now processing well: D17
Now processing well: D18
Now processing well: D19
Now processing well: D20
Now processing well: D21
Now processing well: D22
Now processing well: D23
Now processing well: D24
Now processing well: E01
Now processing well: E02
Now processing well: E03
Now processing well: E04
Now processing well: E05
Now processing well: E06
Now processing well: E07
Now processing well: E08
Now processing well: E09
Now processing well: E10
Now processing well: E11
Now processing well: E12
Now processing well: E13
Now processing well: E14
Now processing well: E15
Now processing well: E16
Now processing well: E17
Now processing well: E18
Now processing well: E19
Now processing well: E20
Now processing well: E21
Now processing well: E22
Now processing well: E23
Now processing well: E24
Now processing well: F01
Now processing well: F02
Now processing well: F03
Now processing well: F04
Now processing well: F05
Now processing well: F06
Now processing well: F13
Now processing well: F14
Now processing well: F15
Now processing well: F16
Now processing well: F17
Now processing well: F18
Now processing well: F19
Now processing well: F20
Now processing well: F21
Now processing well: F22
Now processing well: F23
Now processing well: F24
Now processing well: G01
Now processing well: G02
Now processing well: G03
Now processing well: G04
Now processing well: G05
Now processing well: G06
Now processing well: G07
Now processing well: G08
Now processing well: G09
Now processing well: G10
Now processing well: G11
Now processing well: G12
Now processing well: G19
Now processing well: G20
Now processing well: G21
Now processing well: G22
Now processing well: G23
Now processing well: G24
Now processing well: H01
Now processing well: H02
Now processing well: H03
Now processing well: H04
Now processing well: H05
Now processing well: H06
Now processing well: H07
Now processing well: H08
Now processing well: H09
Now processing well: H10
Now processing well: H11
Now processing well: H12
Now processing well: H13
Now processing well: H14
Now processing well: H15
Now processing well: H16
Now processing well: H17
Now processing well: H18
Now processing well: H19
Now processing well: H20
Now processing well: H21
Now processing well: H22
Now processing well: H23
Now processing well: H24
Now processing well: I01
Now processing well: I02
Now processing well: I03
Now processing well: I04
Now processing well: I05
Now processing well: I06
Now processing well: I07
Now processing well: I08
Now processing well: I09
Now processing well: I10
Now processing well: I11
Now processing well: I12
Now processing well: I13
Now processing well: I14
Now processing well: I15
Now processing well: I16
Now processing well: I17
Now processing well: I18
Now processing well: I19
Now processing well: I20
Now processing well: I21
Now processing well: I22
Now processing well: I23
Now processing well: I24
Now processing well: J01
Now processing well: J02
Now processing well: J03
Now processing well: J04
Now processing well: J05
Now processing well: J06
Now processing well: J07
Now processing well: J08
Now processing well: J09
Now processing well: J10
Now processing well: J11
Now processing well: J12
Now processing well: J19
Now processing well: J20
Now processing well: J21
Now processing well: J22
Now processing well: J23
Now processing well: J24
Now processing well: K01
Now processing well: K02
Now processing well: K03
Now processing well: K04
Now processing well: K05
Now processing well: K06
Now processing well: K07
Now processing well: K08
Now processing well: K09
Now processing well: K10
Now processing well: K11
Now processing well: K12
Now processing well: O01
Now processing well: O02
Now processing well: O03
Now processing well: O04
Now processing well: O05
Now processing well: O06
Now processing well: K13
Now processing well: K14
Now processing well: K15
Now processing well: K16
Now processing well: K17
Now processing well: K18
Now processing well: K19
Now processing well: K20
Now processing well: K21
Now processing well: K22
Now processing well: K23
Now processing well: K24
Now processing well: L01
Now processing well: L02
Now processing well: L03
Now processing well: L04
Now processing well: L05
Now processing well: L06
Now processing well: L07
Now processing well: L08
Now processing well: L09
Now processing well: L10
Now processing well: L11
Now processing well: L12
Now processing well: L13
Now processing well: L14
Now processing well: L15
Now processing well: L16
Now processing well: L17
Now processing well: L18
Now processing well: L19
Now processing well: L20
Now processing well: L21
Now processing well: L22
Now processing well: L23
Now processing well: L24
Now processing well: M01
Now processing well: M02
Now processing well: M03
Now processing well: M04
Now processing well: M05
Now processing well: M06
Now processing well: M07
Now processing well: M08
Now processing well: M09
Now processing well: M10
Now processing well: M11
Now processing well: M12
Now processing well: M13
Now processing well: M14
Now processing well: M15
Now processing well: M16
Now processing well: M17
Now processing well: M18
Now processing well: M19
Now processing well: M20
Now processing well: M21
Now processing well: M22
Now processing well: M23
Now processing well: M24
Now processing well: N01
Now processing well: N02
Now processing well: N03
Now processing well: N04
Now processing well: N05
Now processing well: N06
Now processing well: N07
Now processing well: N08
Now processing well: N09
Now processing well: N10
Now processing well: N11
Now processing well: N12
Now processing well: N13
Now processing well: N14
Now processing well: N15
Now processing well: N16
Now processing well: N17
Now processing well: N18
Now processing well: N19
Now processing well: N20
Now processing well: N21
Now processing well: N22
Now processing well: N23
Now processing well: N24
Now processing well: O07
Now processing well: O08
Now processing well: O09
Now processing well: O10
Now processing well: O11
Now processing well: O12
Now processing well: O13
Now processing well: O14
Now processing well: O15
Now processing well: O16
Now processing well: O17
Now processing well: O18
Now processing well: O19
Now processing well: O20
Now processing well: O21
Now processing well: O22
Now processing well: O23
Now processing well: O24
Now processing well: P01
Now processing well: P02
Now processing well: P03
Now processing well: P04
Now processing well: P05
Now processing well: P06
Now processing well: P07
Now processing well: P08
Now processing well: P09
Now processing well: P10
Now processing well: P11
Now processing well: P12
Now processing well: P13
Now processing well: P14
Now processing well: P15
Now processing well: P16
Now processing well: P17
Now processing well: P18
Now processing well: P19
Now processing well: P20
Now processing well: P21
Now processing well: P22
Now processing well: P23
Now processing well: P24
###Markdown
Calculate non-parametric p value
###Code
non_parametric_p_vals = []
for idx, treatment in df_cpd_med_scores_melt.iterrows():
compound = treatment.compound
cardinality = treatment.no_of_replicates
if cardinality == 1:
continue
well = treatment.Metadata_Well
dose = treatment.dose_recode
score = treatment.median_score
# Pull appropriate null distribution:
null_dist = full_well_null_distribution_of_scores[well][cardinality]
# Calculate p value
p_value = np.sum(np.less(score, null_dist)) / len(null_dist)
# Save results
results = [
compound,
cardinality,
well,
dose,
score,
p_value
]
# Add to list
non_parametric_p_vals.append(results)
# Get full results
pval_df = pd.DataFrame(
non_parametric_p_vals,
columns=[
"compound",
"no_of_compounds",
"well",
"dose_recode",
"median_score",
"p_value"
]
)
# Save to file
pval_df.to_csv(output_file, index=False, sep="\t")
print(pval_df.shape)
pval_df.head()
###Output
(7572, 6)
|
src_data/20191022_deltaescv_eyfp-escv_aht20/04_optimization_and_selection.ipynb | ###Markdown
epec_yfp-escv_aht20
###Code
f = 'epec_yfp-escv_aht20'
bn = ~np.equal([r for r in gd[f].res], None)
len(bn) - bn.sum()
gd[f].obj = np.array([r.objective_value for r in np.array(gd[f].res)[bn]])
gd[f].area = np.array([c.data.binary_img.sum() for c in gd[f].bin_cells[bn]])
ratio = gd[f].obj / gd[f].area
plt.figure()
h = plt.hist(ratio, bins='fd')
b = ratio < 0.1
aicp = AutoIterCellPlot(gd[f].bin_cells[bn][b])
aicp.plot()
gd[f].selected = gd[f].bin_cells[bn][b]
save(f + '_binary_opt.hdf5', gd[f].selected)
###Output
_____no_output_____ |
tasks/19/Task-Count of injured.ipynb | ###Markdown
Count of injured: per injury severity
###Code
print(involved_hebrew['injury_severity'].isnull().sum())
print(involved_hebrew['injury_severity'].value_counts())
sns.countplot(x='injury_severity', data=involved_hebrew)
# Count of injured per injury severity
total_accidents = involved_hebrew.shape[0]
injury_severity_percentage = involved_hebrew.groupby('injury_severity')['accident_id'].count() / total_accidents
print(injury_severity_percentage)
sns.barplot(x=injury_severity_percentage.index, y=injury_severity_percentage)
# Count of injured per injury severity normalized
###Output
injury_severity
0 0.375925
1 0.002293
2 0.012532
3 0.609250
Name: accident_id, dtype: float64
###Markdown
Count of injured: inter/in-city
###Code
markers_hebrew = pd.read_csv('../data/views_2019/views_2019/markers_hebrew.csv')
markers_hebrew.head().T
markers_hebrew['road_type'].head()
# 1,2 -> In-city, 3,4 -> Inter-city
# roads are only for inter-city data records
# while streets are only for in-city
def inter_or_in_city(road_type):
# 0 is in-city, 1 is inter-city
if road_type == 1 or road_type == 2:
return 0
else:
return 1
markers_hebrew['inter/in-city'] = markers_hebrew['road_type'].apply(inter_or_in_city)
markers_hebrew['inter/in-city'].head()
sns.countplot(x='inter/in-city', data=markers_hebrew)
# Count of injured per inter/in-city
total_accidents = markers_hebrew.shape[0]
distr_percentage = markers_hebrew.groupby('inter/in-city')['provider_and_id'].count() / total_accidents
print(distr_percentage)
sns.barplot(x=distr_percentage.index, y=distr_percentage)
# Count of injured per inter/in-city normalized
###Output
inter/in-city
0 0.751402
1 0.248598
Name: provider_and_id, dtype: float64
###Markdown
Count of injured: per geo resolution/radius
###Code
news_flash = pd.read_csv('../data/news_flash.csv')
news_flash.head().T
def plot_based_on_radius(accident_df, coordinates):
# Accepts input (lat, lng) to be used as the center of the radius
###Output
_____no_output_____ |
4_de_analysis/1_de_control.ipynb | ###Markdown
Differential expression analysis controlAs a way of quantifying how similar/different the expression profiles associated with different mutation patterns are, we want to count the number of differentially expressed genes between them. To make sure this makes sense, we first want to take some cancer subtypes we know are quite different, and compare the number of DE genes between them to the number of DE genes between random samples of the same size.We expect to see that different subtypes have considerably more DE genes between them than random samples taken uniformly from the different subtypes.
###Code
from pathlib import Path
import pickle as pkl
import pandas as pd
import sys; sys.path.append('..')
import config as cfg
%load_ext autoreload
%autoreload 2
# if True rerun DE analysis and overwrite existing results
# if False look for existing results and don't rerun DE analysis
# (the latter makes the notebook run much faster)
RUN_DE_ANALYSIS = False
###Output
_____no_output_____
###Markdown
Load datasets
###Code
# load counts data
all_counts_df = pd.read_csv(cfg.processed_counts_file, sep='\t', index_col=0)
print(all_counts_df.shape)
all_counts_df.iloc[:5, :5]
# load cancer types
sample_info_df = pd.read_csv(cfg.de_sample_info, sep='\t', index_col=0)
print(sample_info_df.shape)
sample_info_df.head()
# load mutation status
pancancer_pickle = Path('/home/jake/research/mpmp/data/pancancer_data.pkl')
with open(pancancer_pickle, 'rb') as f:
pancancer_data = pkl.load(f)
mutation_df = pancancer_data[1]
print(mutation_df.shape)
mutation_df.iloc[:5, :5]
###Output
(9074, 20938)
###Markdown
DE between IDH1 mutant/wild-type samples in low-grade glioma
###Code
cfg.de_input_dir.mkdir(parents=True, exist_ok=True)
cfg.de_output_dir.mkdir(parents=True, exist_ok=True)
base_dir = str(cfg.de_base_dir)
output_dir = str(cfg.de_output_dir)
# get LGG samples from counts data
lgg_samples = (
sample_info_df[sample_info_df.cancer_type == 'LGG'].index
.intersection(all_counts_df.index)
.intersection(mutation_df.index)
)
lgg_counts_df = all_counts_df.loc[lgg_samples, :]
print(lgg_counts_df.shape)
lgg_counts_df.iloc[:5, :5]
# save LGG samples to file, to be loaded by DESeq2
input_file = cfg.de_input_dir / 'lgg_counts.tsv'
input_str = str(input_file)
lgg_counts_df.to_csv(input_file, sep='\t')
# get IDH1 mutation status
idh1_status_df = (mutation_df
.loc[lgg_samples, ['IDH1']]
.rename(columns={'IDH1': 'group'})
)
idh1_status_df.head()
# save mutation status to file, to be loaded by DESeq2
input_metadata_file = cfg.de_input_dir / 'lgg_idh1_status.tsv'
input_metadata_str = str(input_metadata_file)
idh1_status_df.to_csv(input_metadata_file, sep='\t')
%load_ext rpy2.ipython
%%R -i RUN_DE_ANALYSIS -i base_dir -i input_metadata_str -i input_str -i output_dir
if (RUN_DE_ANALYSIS) {
source(paste0(base_dir, '/de_analysis.R'))
get_DE_stats_DESeq(input_metadata_str,
input_str,
'LGG_IDH1',
output_dir)
} else {
print('Skipping DE analysis, will use existing results files')
}
###Output
[1] "Skipping DE analysis, will use existing results files"
###Markdown
DE between random samples in low-grade gliomaWe do this to generate an empirical null distribution for our results in IDH1 mutants/wild-type samples.
###Code
# number of random samples
n_samples = 5
n_mutated = idh1_status_df.sum().values[0]
n_not_mutated = idh1_status_df.shape[0] - n_mutated
print(n_mutated, n_not_mutated)
# we can use sklearn train_test_split to partition the data randomly
import numpy as np
from sklearn.model_selection import train_test_split
for sample_ix in range(n_samples):
_, test_ixs = train_test_split(idh1_status_df.index,
test_size=n_mutated,
shuffle=True,
random_state=sample_ix)
labels_df = pd.DataFrame(
np.zeros(idh1_status_df.shape[0]).astype(int),
index=idh1_status_df.index.copy(),
columns=['group']
)
labels_df.loc[test_ixs, 'group'] = 1
save_file = cfg.de_input_dir / 'lgg_idh1_random_s{}.tsv'.format(sample_ix)
print(str(save_file))
labels_df.to_csv(save_file, sep='\t')
input_metadata_dir = str(cfg.de_input_dir)
%%R -i RUN_DE_ANALYSIS -i base_dir -i input_str -i n_samples -i input_metadata_dir -i output_dir
if (RUN_DE_ANALYSIS) {
source(paste0(base_dir, '/de_analysis.R'))
for (i in 0:(n_samples-1)) {
print(paste('Running: ', i))
input_metadata_str <- paste(
input_metadata_dir, '/lgg_idh1_random_s', i, '.tsv',
sep=''
)
get_DE_stats_DESeq(input_metadata_str,
input_str,
paste('LGG_IDH1_random_s', i, sep=''),
output_dir)
}
} else {
print('Skipping DE analysis, will use existing results files')
}
###Output
[1] "Skipping DE analysis, will use existing results files"
###Markdown
Compare IDH1 mutation DE results to randomly sampled results
###Code
idh1_de_results_df = pd.read_csv(
cfg.de_output_dir / 'DE_stats_LGG_IDH1.txt',
sep='\t'
)
print(idh1_de_results_df.shape)
idh1_de_results_df.head()
random_de_results = []
for i in range(n_samples):
random_de_results.append(
pd.read_csv(
cfg.de_output_dir / 'DE_stats_LGG_IDH1_random_s{}.txt'.format(i),
sep='\t'
)
)
print(random_de_results[0].shape)
random_de_results[0].head()
# adjusted p-value threshold
alpha = 0.05
idh1_de_count = (
(idh1_de_results_df.padj < alpha).sum()
)
random_de_count = [
(random_de_results[ix].padj < alpha).sum() for ix in range(n_samples)
]
print('DE genes for IDH1 WT vs. mutant:', idh1_de_count)
print('DE genes for random size-matched samples:', random_de_count)
import matplotlib.pyplot as plt
import seaborn as sns
sns.set({'figure.figsize': (8, 6)})
sns.kdeplot(data=idh1_de_results_df.pvalue, label='true')
for ix in range(n_samples):
if ix == 0:
sns.kdeplot(data=random_de_results[ix].pvalue,
color='red', label='random')
else:
sns.kdeplot(data=random_de_results[ix].pvalue, color='red')
plt.title('Uncorrected p-value density distributions')
plt.xlabel('uncorrected p-value')
plt.legend()
import matplotlib.pyplot as plt
import seaborn as sns
sns.set({'figure.figsize': (8, 6)})
sns.kdeplot(data=idh1_de_results_df.padj, label='true')
for ix in range(n_samples):
if ix == 0:
sns.kdeplot(data=random_de_results[ix].padj,
color='red', label='random')
else:
sns.kdeplot(data=random_de_results[ix].padj, color='red')
plt.title('FDR corrected p-value density distributions')
plt.xlabel('Corrected p-value')
plt.legend()
###Output
_____no_output_____ |
day4/.ipynb_checkpoints/day4-checkpoint.ipynb | ###Markdown
end data preparation, the new data represent the item who has been bought together.
###Code
basket.head()
def encode_units(x):
if x <= 0:
return 0
if x >= 1:
return 1
basket_sets = basket.applymap(encode_units)
basket_sets.drop('POSTAGE', inplace=True, axis=1)
frequent_itemsets = apriori(basket_sets, min_support=0.07, use_colnames=True)
rules = association_rules(frequent_itemsets, metric="lift", min_threshold=1)
rules[ (rules['lift'] >= 6) &
(rules['confidence'] >= 0.8) ]
###Output
_____no_output_____ |
Model backlog/Models/Inference/52-cassava-leaf-inf-effnetb5-step-200-bs-8-512.ipynb | ###Markdown
Dependencies
###Code
!pip install --quiet /kaggle/input/kerasapplications
!pip install --quiet /kaggle/input/efficientnet-git
import warnings, glob
from tensorflow.keras import Sequential, Model
import efficientnet.tfkeras as efn
from cassava_scripts import *
seed = 0
seed_everything(seed)
warnings.filterwarnings('ignore')
###Output
_____no_output_____
###Markdown
Hardware configuration
###Code
# TPU or GPU detection
# Detect hardware, return appropriate distribution strategy
strategy, tpu = set_up_strategy()
AUTO = tf.data.experimental.AUTOTUNE
REPLICAS = strategy.num_replicas_in_sync
print(f'REPLICAS: {REPLICAS}')
###Output
REPLICAS: 1
###Markdown
Model parameters
###Code
BATCH_SIZE = 8 * REPLICAS
HEIGHT = 512
WIDTH = 512
CHANNELS = 3
N_CLASSES = 5
TTA_STEPS = 0 # Do TTA if > 0
###Output
_____no_output_____
###Markdown
Augmentation
###Code
def data_augment(image, label):
p_spatial = tf.random.uniform([], 0, 1.0, dtype=tf.float32)
p_rotate = tf.random.uniform([], 0, 1.0, dtype=tf.float32)
p_pixel_1 = tf.random.uniform([], 0, 1.0, dtype=tf.float32)
p_pixel_2 = tf.random.uniform([], 0, 1.0, dtype=tf.float32)
p_pixel_3 = tf.random.uniform([], 0, 1.0, dtype=tf.float32)
p_crop = tf.random.uniform([], 0, 1.0, dtype=tf.float32)
# Flips
image = tf.image.random_flip_left_right(image)
image = tf.image.random_flip_up_down(image)
if p_spatial > .75:
image = tf.image.transpose(image)
# Rotates
if p_rotate > .75:
image = tf.image.rot90(image, k=3) # rotate 270º
elif p_rotate > .5:
image = tf.image.rot90(image, k=2) # rotate 180º
elif p_rotate > .25:
image = tf.image.rot90(image, k=1) # rotate 90º
# Pixel-level transforms
if p_pixel_1 >= .4:
image = tf.image.random_saturation(image, lower=.7, upper=1.3)
if p_pixel_2 >= .4:
image = tf.image.random_contrast(image, lower=.8, upper=1.2)
if p_pixel_3 >= .4:
image = tf.image.random_brightness(image, max_delta=.1)
# Crops
if p_crop > .7:
if p_crop > .9:
image = tf.image.central_crop(image, central_fraction=.7)
elif p_crop > .8:
image = tf.image.central_crop(image, central_fraction=.8)
else:
image = tf.image.central_crop(image, central_fraction=.9)
elif p_crop > .4:
crop_size = tf.random.uniform([], int(HEIGHT*.8), HEIGHT, dtype=tf.int32)
image = tf.image.random_crop(image, size=[crop_size, crop_size, CHANNELS])
# # Crops
# if p_crop > .6:
# if p_crop > .9:
# image = tf.image.central_crop(image, central_fraction=.5)
# elif p_crop > .8:
# image = tf.image.central_crop(image, central_fraction=.6)
# elif p_crop > .7:
# image = tf.image.central_crop(image, central_fraction=.7)
# else:
# image = tf.image.central_crop(image, central_fraction=.8)
# elif p_crop > .3:
# crop_size = tf.random.uniform([], int(HEIGHT*.6), HEIGHT, dtype=tf.int32)
# image = tf.image.random_crop(image, size=[crop_size, crop_size, CHANNELS])
return image, label
###Output
_____no_output_____
###Markdown
Auxiliary functions
###Code
# Datasets utility functions
def resize_image(image, label):
image = tf.image.resize(image, [HEIGHT, WIDTH])
image = tf.reshape(image, [HEIGHT, WIDTH, CHANNELS])
return image, label
def process_path(file_path):
name = get_name(file_path)
img = tf.io.read_file(file_path)
img = decode_image(img)
img, _ = scale_image(img, None)
# img = center_crop(img, HEIGHT, WIDTH)
return img, name
def get_dataset(files_path, shuffled=False, tta=False, extension='jpg'):
dataset = tf.data.Dataset.list_files(f'{files_path}*{extension}', shuffle=shuffled)
dataset = dataset.map(process_path, num_parallel_calls=AUTO)
if tta:
dataset = dataset.map(data_augment, num_parallel_calls=AUTO)
dataset = dataset.map(resize_image, num_parallel_calls=AUTO)
dataset = dataset.batch(BATCH_SIZE)
dataset = dataset.prefetch(AUTO)
return dataset
###Output
_____no_output_____
###Markdown
Load data
###Code
database_base_path = '/kaggle/input/cassava-leaf-disease-classification/'
submission = pd.read_csv(f'{database_base_path}sample_submission.csv')
display(submission.head())
TEST_FILENAMES = tf.io.gfile.glob(f'{database_base_path}test_tfrecords/ld_test*.tfrec')
NUM_TEST_IMAGES = count_data_items(TEST_FILENAMES)
print(f'GCS: test: {NUM_TEST_IMAGES}')
!ls /kaggle/input/
model_path_list = glob.glob('/kaggle/input/52-cassava-leaf-effnetb5-step-200-bs-8-512/*.h5')
model_path_list.sort()
print('Models to predict:')
print(*model_path_list, sep='\n')
###Output
Models to predict:
/kaggle/input/52-cassava-leaf-effnetb5-step-200-bs-8-512/model_0.h5
###Markdown
Model
###Code
def model_fn(input_shape, N_CLASSES):
inputs = L.Input(shape=input_shape, name='input_image')
base_model = efn.EfficientNetB5(input_tensor=inputs,
include_top=False,
weights=None,
pooling='avg')
x = L.Dropout(.25)(base_model.output)
output = L.Dense(N_CLASSES, activation='softmax', name='output')(x)
model = Model(inputs=inputs, outputs=output)
return model
with strategy.scope():
model = model_fn((None, None, CHANNELS), N_CLASSES)
model.summary()
###Output
Model: "model"
__________________________________________________________________________________________________
Layer (type) Output Shape Param # Connected to
==================================================================================================
input_image (InputLayer) [(None, None, None, 0
__________________________________________________________________________________________________
stem_conv (Conv2D) (None, None, None, 4 1296 input_image[0][0]
__________________________________________________________________________________________________
stem_bn (BatchNormalization) (None, None, None, 4 192 stem_conv[0][0]
__________________________________________________________________________________________________
stem_activation (Activation) (None, None, None, 4 0 stem_bn[0][0]
__________________________________________________________________________________________________
block1a_dwconv (DepthwiseConv2D (None, None, None, 4 432 stem_activation[0][0]
__________________________________________________________________________________________________
block1a_bn (BatchNormalization) (None, None, None, 4 192 block1a_dwconv[0][0]
__________________________________________________________________________________________________
block1a_activation (Activation) (None, None, None, 4 0 block1a_bn[0][0]
__________________________________________________________________________________________________
block1a_se_squeeze (GlobalAvera (None, 48) 0 block1a_activation[0][0]
__________________________________________________________________________________________________
block1a_se_reshape (Reshape) (None, 1, 1, 48) 0 block1a_se_squeeze[0][0]
__________________________________________________________________________________________________
block1a_se_reduce (Conv2D) (None, 1, 1, 12) 588 block1a_se_reshape[0][0]
__________________________________________________________________________________________________
block1a_se_expand (Conv2D) (None, 1, 1, 48) 624 block1a_se_reduce[0][0]
__________________________________________________________________________________________________
block1a_se_excite (Multiply) (None, None, None, 4 0 block1a_activation[0][0]
block1a_se_expand[0][0]
__________________________________________________________________________________________________
block1a_project_conv (Conv2D) (None, None, None, 2 1152 block1a_se_excite[0][0]
__________________________________________________________________________________________________
block1a_project_bn (BatchNormal (None, None, None, 2 96 block1a_project_conv[0][0]
__________________________________________________________________________________________________
block1b_dwconv (DepthwiseConv2D (None, None, None, 2 216 block1a_project_bn[0][0]
__________________________________________________________________________________________________
block1b_bn (BatchNormalization) (None, None, None, 2 96 block1b_dwconv[0][0]
__________________________________________________________________________________________________
block1b_activation (Activation) (None, None, None, 2 0 block1b_bn[0][0]
__________________________________________________________________________________________________
block1b_se_squeeze (GlobalAvera (None, 24) 0 block1b_activation[0][0]
__________________________________________________________________________________________________
block1b_se_reshape (Reshape) (None, 1, 1, 24) 0 block1b_se_squeeze[0][0]
__________________________________________________________________________________________________
block1b_se_reduce (Conv2D) (None, 1, 1, 6) 150 block1b_se_reshape[0][0]
__________________________________________________________________________________________________
block1b_se_expand (Conv2D) (None, 1, 1, 24) 168 block1b_se_reduce[0][0]
__________________________________________________________________________________________________
block1b_se_excite (Multiply) (None, None, None, 2 0 block1b_activation[0][0]
block1b_se_expand[0][0]
__________________________________________________________________________________________________
block1b_project_conv (Conv2D) (None, None, None, 2 576 block1b_se_excite[0][0]
__________________________________________________________________________________________________
block1b_project_bn (BatchNormal (None, None, None, 2 96 block1b_project_conv[0][0]
__________________________________________________________________________________________________
block1b_drop (FixedDropout) (None, None, None, 2 0 block1b_project_bn[0][0]
__________________________________________________________________________________________________
block1b_add (Add) (None, None, None, 2 0 block1b_drop[0][0]
block1a_project_bn[0][0]
__________________________________________________________________________________________________
block1c_dwconv (DepthwiseConv2D (None, None, None, 2 216 block1b_add[0][0]
__________________________________________________________________________________________________
block1c_bn (BatchNormalization) (None, None, None, 2 96 block1c_dwconv[0][0]
__________________________________________________________________________________________________
block1c_activation (Activation) (None, None, None, 2 0 block1c_bn[0][0]
__________________________________________________________________________________________________
block1c_se_squeeze (GlobalAvera (None, 24) 0 block1c_activation[0][0]
__________________________________________________________________________________________________
block1c_se_reshape (Reshape) (None, 1, 1, 24) 0 block1c_se_squeeze[0][0]
__________________________________________________________________________________________________
block1c_se_reduce (Conv2D) (None, 1, 1, 6) 150 block1c_se_reshape[0][0]
__________________________________________________________________________________________________
block1c_se_expand (Conv2D) (None, 1, 1, 24) 168 block1c_se_reduce[0][0]
__________________________________________________________________________________________________
block1c_se_excite (Multiply) (None, None, None, 2 0 block1c_activation[0][0]
block1c_se_expand[0][0]
__________________________________________________________________________________________________
block1c_project_conv (Conv2D) (None, None, None, 2 576 block1c_se_excite[0][0]
__________________________________________________________________________________________________
block1c_project_bn (BatchNormal (None, None, None, 2 96 block1c_project_conv[0][0]
__________________________________________________________________________________________________
block1c_drop (FixedDropout) (None, None, None, 2 0 block1c_project_bn[0][0]
__________________________________________________________________________________________________
block1c_add (Add) (None, None, None, 2 0 block1c_drop[0][0]
block1b_add[0][0]
__________________________________________________________________________________________________
block2a_expand_conv (Conv2D) (None, None, None, 1 3456 block1c_add[0][0]
__________________________________________________________________________________________________
block2a_expand_bn (BatchNormali (None, None, None, 1 576 block2a_expand_conv[0][0]
__________________________________________________________________________________________________
block2a_expand_activation (Acti (None, None, None, 1 0 block2a_expand_bn[0][0]
__________________________________________________________________________________________________
block2a_dwconv (DepthwiseConv2D (None, None, None, 1 1296 block2a_expand_activation[0][0]
__________________________________________________________________________________________________
block2a_bn (BatchNormalization) (None, None, None, 1 576 block2a_dwconv[0][0]
__________________________________________________________________________________________________
block2a_activation (Activation) (None, None, None, 1 0 block2a_bn[0][0]
__________________________________________________________________________________________________
block2a_se_squeeze (GlobalAvera (None, 144) 0 block2a_activation[0][0]
__________________________________________________________________________________________________
block2a_se_reshape (Reshape) (None, 1, 1, 144) 0 block2a_se_squeeze[0][0]
__________________________________________________________________________________________________
block2a_se_reduce (Conv2D) (None, 1, 1, 6) 870 block2a_se_reshape[0][0]
__________________________________________________________________________________________________
block2a_se_expand (Conv2D) (None, 1, 1, 144) 1008 block2a_se_reduce[0][0]
__________________________________________________________________________________________________
block2a_se_excite (Multiply) (None, None, None, 1 0 block2a_activation[0][0]
block2a_se_expand[0][0]
__________________________________________________________________________________________________
block2a_project_conv (Conv2D) (None, None, None, 4 5760 block2a_se_excite[0][0]
__________________________________________________________________________________________________
block2a_project_bn (BatchNormal (None, None, None, 4 160 block2a_project_conv[0][0]
__________________________________________________________________________________________________
block2b_expand_conv (Conv2D) (None, None, None, 2 9600 block2a_project_bn[0][0]
__________________________________________________________________________________________________
block2b_expand_bn (BatchNormali (None, None, None, 2 960 block2b_expand_conv[0][0]
__________________________________________________________________________________________________
block2b_expand_activation (Acti (None, None, None, 2 0 block2b_expand_bn[0][0]
__________________________________________________________________________________________________
block2b_dwconv (DepthwiseConv2D (None, None, None, 2 2160 block2b_expand_activation[0][0]
__________________________________________________________________________________________________
block2b_bn (BatchNormalization) (None, None, None, 2 960 block2b_dwconv[0][0]
__________________________________________________________________________________________________
block2b_activation (Activation) (None, None, None, 2 0 block2b_bn[0][0]
__________________________________________________________________________________________________
block2b_se_squeeze (GlobalAvera (None, 240) 0 block2b_activation[0][0]
__________________________________________________________________________________________________
block2b_se_reshape (Reshape) (None, 1, 1, 240) 0 block2b_se_squeeze[0][0]
__________________________________________________________________________________________________
block2b_se_reduce (Conv2D) (None, 1, 1, 10) 2410 block2b_se_reshape[0][0]
__________________________________________________________________________________________________
block2b_se_expand (Conv2D) (None, 1, 1, 240) 2640 block2b_se_reduce[0][0]
__________________________________________________________________________________________________
block2b_se_excite (Multiply) (None, None, None, 2 0 block2b_activation[0][0]
block2b_se_expand[0][0]
__________________________________________________________________________________________________
block2b_project_conv (Conv2D) (None, None, None, 4 9600 block2b_se_excite[0][0]
__________________________________________________________________________________________________
block2b_project_bn (BatchNormal (None, None, None, 4 160 block2b_project_conv[0][0]
__________________________________________________________________________________________________
block2b_drop (FixedDropout) (None, None, None, 4 0 block2b_project_bn[0][0]
__________________________________________________________________________________________________
block2b_add (Add) (None, None, None, 4 0 block2b_drop[0][0]
block2a_project_bn[0][0]
__________________________________________________________________________________________________
block2c_expand_conv (Conv2D) (None, None, None, 2 9600 block2b_add[0][0]
__________________________________________________________________________________________________
block2c_expand_bn (BatchNormali (None, None, None, 2 960 block2c_expand_conv[0][0]
__________________________________________________________________________________________________
block2c_expand_activation (Acti (None, None, None, 2 0 block2c_expand_bn[0][0]
__________________________________________________________________________________________________
block2c_dwconv (DepthwiseConv2D (None, None, None, 2 2160 block2c_expand_activation[0][0]
__________________________________________________________________________________________________
block2c_bn (BatchNormalization) (None, None, None, 2 960 block2c_dwconv[0][0]
__________________________________________________________________________________________________
block2c_activation (Activation) (None, None, None, 2 0 block2c_bn[0][0]
__________________________________________________________________________________________________
block2c_se_squeeze (GlobalAvera (None, 240) 0 block2c_activation[0][0]
__________________________________________________________________________________________________
block2c_se_reshape (Reshape) (None, 1, 1, 240) 0 block2c_se_squeeze[0][0]
__________________________________________________________________________________________________
block2c_se_reduce (Conv2D) (None, 1, 1, 10) 2410 block2c_se_reshape[0][0]
__________________________________________________________________________________________________
block2c_se_expand (Conv2D) (None, 1, 1, 240) 2640 block2c_se_reduce[0][0]
__________________________________________________________________________________________________
block2c_se_excite (Multiply) (None, None, None, 2 0 block2c_activation[0][0]
block2c_se_expand[0][0]
__________________________________________________________________________________________________
block2c_project_conv (Conv2D) (None, None, None, 4 9600 block2c_se_excite[0][0]
__________________________________________________________________________________________________
block2c_project_bn (BatchNormal (None, None, None, 4 160 block2c_project_conv[0][0]
__________________________________________________________________________________________________
block2c_drop (FixedDropout) (None, None, None, 4 0 block2c_project_bn[0][0]
__________________________________________________________________________________________________
block2c_add (Add) (None, None, None, 4 0 block2c_drop[0][0]
block2b_add[0][0]
__________________________________________________________________________________________________
block2d_expand_conv (Conv2D) (None, None, None, 2 9600 block2c_add[0][0]
__________________________________________________________________________________________________
block2d_expand_bn (BatchNormali (None, None, None, 2 960 block2d_expand_conv[0][0]
__________________________________________________________________________________________________
block2d_expand_activation (Acti (None, None, None, 2 0 block2d_expand_bn[0][0]
__________________________________________________________________________________________________
block2d_dwconv (DepthwiseConv2D (None, None, None, 2 2160 block2d_expand_activation[0][0]
__________________________________________________________________________________________________
block2d_bn (BatchNormalization) (None, None, None, 2 960 block2d_dwconv[0][0]
__________________________________________________________________________________________________
block2d_activation (Activation) (None, None, None, 2 0 block2d_bn[0][0]
__________________________________________________________________________________________________
block2d_se_squeeze (GlobalAvera (None, 240) 0 block2d_activation[0][0]
__________________________________________________________________________________________________
block2d_se_reshape (Reshape) (None, 1, 1, 240) 0 block2d_se_squeeze[0][0]
__________________________________________________________________________________________________
block2d_se_reduce (Conv2D) (None, 1, 1, 10) 2410 block2d_se_reshape[0][0]
__________________________________________________________________________________________________
block2d_se_expand (Conv2D) (None, 1, 1, 240) 2640 block2d_se_reduce[0][0]
__________________________________________________________________________________________________
block2d_se_excite (Multiply) (None, None, None, 2 0 block2d_activation[0][0]
block2d_se_expand[0][0]
__________________________________________________________________________________________________
block2d_project_conv (Conv2D) (None, None, None, 4 9600 block2d_se_excite[0][0]
__________________________________________________________________________________________________
block2d_project_bn (BatchNormal (None, None, None, 4 160 block2d_project_conv[0][0]
__________________________________________________________________________________________________
block2d_drop (FixedDropout) (None, None, None, 4 0 block2d_project_bn[0][0]
__________________________________________________________________________________________________
block2d_add (Add) (None, None, None, 4 0 block2d_drop[0][0]
block2c_add[0][0]
__________________________________________________________________________________________________
block2e_expand_conv (Conv2D) (None, None, None, 2 9600 block2d_add[0][0]
__________________________________________________________________________________________________
block2e_expand_bn (BatchNormali (None, None, None, 2 960 block2e_expand_conv[0][0]
__________________________________________________________________________________________________
block2e_expand_activation (Acti (None, None, None, 2 0 block2e_expand_bn[0][0]
__________________________________________________________________________________________________
block2e_dwconv (DepthwiseConv2D (None, None, None, 2 2160 block2e_expand_activation[0][0]
__________________________________________________________________________________________________
block2e_bn (BatchNormalization) (None, None, None, 2 960 block2e_dwconv[0][0]
__________________________________________________________________________________________________
block2e_activation (Activation) (None, None, None, 2 0 block2e_bn[0][0]
__________________________________________________________________________________________________
block2e_se_squeeze (GlobalAvera (None, 240) 0 block2e_activation[0][0]
__________________________________________________________________________________________________
block2e_se_reshape (Reshape) (None, 1, 1, 240) 0 block2e_se_squeeze[0][0]
__________________________________________________________________________________________________
block2e_se_reduce (Conv2D) (None, 1, 1, 10) 2410 block2e_se_reshape[0][0]
__________________________________________________________________________________________________
block2e_se_expand (Conv2D) (None, 1, 1, 240) 2640 block2e_se_reduce[0][0]
__________________________________________________________________________________________________
block2e_se_excite (Multiply) (None, None, None, 2 0 block2e_activation[0][0]
block2e_se_expand[0][0]
__________________________________________________________________________________________________
block2e_project_conv (Conv2D) (None, None, None, 4 9600 block2e_se_excite[0][0]
__________________________________________________________________________________________________
block2e_project_bn (BatchNormal (None, None, None, 4 160 block2e_project_conv[0][0]
__________________________________________________________________________________________________
block2e_drop (FixedDropout) (None, None, None, 4 0 block2e_project_bn[0][0]
__________________________________________________________________________________________________
block2e_add (Add) (None, None, None, 4 0 block2e_drop[0][0]
block2d_add[0][0]
__________________________________________________________________________________________________
block3a_expand_conv (Conv2D) (None, None, None, 2 9600 block2e_add[0][0]
__________________________________________________________________________________________________
block3a_expand_bn (BatchNormali (None, None, None, 2 960 block3a_expand_conv[0][0]
__________________________________________________________________________________________________
block3a_expand_activation (Acti (None, None, None, 2 0 block3a_expand_bn[0][0]
__________________________________________________________________________________________________
block3a_dwconv (DepthwiseConv2D (None, None, None, 2 6000 block3a_expand_activation[0][0]
__________________________________________________________________________________________________
block3a_bn (BatchNormalization) (None, None, None, 2 960 block3a_dwconv[0][0]
__________________________________________________________________________________________________
block3a_activation (Activation) (None, None, None, 2 0 block3a_bn[0][0]
__________________________________________________________________________________________________
block3a_se_squeeze (GlobalAvera (None, 240) 0 block3a_activation[0][0]
__________________________________________________________________________________________________
block3a_se_reshape (Reshape) (None, 1, 1, 240) 0 block3a_se_squeeze[0][0]
__________________________________________________________________________________________________
block3a_se_reduce (Conv2D) (None, 1, 1, 10) 2410 block3a_se_reshape[0][0]
__________________________________________________________________________________________________
block3a_se_expand (Conv2D) (None, 1, 1, 240) 2640 block3a_se_reduce[0][0]
__________________________________________________________________________________________________
block3a_se_excite (Multiply) (None, None, None, 2 0 block3a_activation[0][0]
block3a_se_expand[0][0]
__________________________________________________________________________________________________
block3a_project_conv (Conv2D) (None, None, None, 6 15360 block3a_se_excite[0][0]
__________________________________________________________________________________________________
block3a_project_bn (BatchNormal (None, None, None, 6 256 block3a_project_conv[0][0]
__________________________________________________________________________________________________
block3b_expand_conv (Conv2D) (None, None, None, 3 24576 block3a_project_bn[0][0]
__________________________________________________________________________________________________
block3b_expand_bn (BatchNormali (None, None, None, 3 1536 block3b_expand_conv[0][0]
__________________________________________________________________________________________________
block3b_expand_activation (Acti (None, None, None, 3 0 block3b_expand_bn[0][0]
__________________________________________________________________________________________________
block3b_dwconv (DepthwiseConv2D (None, None, None, 3 9600 block3b_expand_activation[0][0]
__________________________________________________________________________________________________
block3b_bn (BatchNormalization) (None, None, None, 3 1536 block3b_dwconv[0][0]
__________________________________________________________________________________________________
block3b_activation (Activation) (None, None, None, 3 0 block3b_bn[0][0]
__________________________________________________________________________________________________
block3b_se_squeeze (GlobalAvera (None, 384) 0 block3b_activation[0][0]
__________________________________________________________________________________________________
block3b_se_reshape (Reshape) (None, 1, 1, 384) 0 block3b_se_squeeze[0][0]
__________________________________________________________________________________________________
block3b_se_reduce (Conv2D) (None, 1, 1, 16) 6160 block3b_se_reshape[0][0]
__________________________________________________________________________________________________
block3b_se_expand (Conv2D) (None, 1, 1, 384) 6528 block3b_se_reduce[0][0]
__________________________________________________________________________________________________
block3b_se_excite (Multiply) (None, None, None, 3 0 block3b_activation[0][0]
block3b_se_expand[0][0]
__________________________________________________________________________________________________
block3b_project_conv (Conv2D) (None, None, None, 6 24576 block3b_se_excite[0][0]
__________________________________________________________________________________________________
block3b_project_bn (BatchNormal (None, None, None, 6 256 block3b_project_conv[0][0]
__________________________________________________________________________________________________
block3b_drop (FixedDropout) (None, None, None, 6 0 block3b_project_bn[0][0]
__________________________________________________________________________________________________
block3b_add (Add) (None, None, None, 6 0 block3b_drop[0][0]
block3a_project_bn[0][0]
__________________________________________________________________________________________________
block3c_expand_conv (Conv2D) (None, None, None, 3 24576 block3b_add[0][0]
__________________________________________________________________________________________________
block3c_expand_bn (BatchNormali (None, None, None, 3 1536 block3c_expand_conv[0][0]
__________________________________________________________________________________________________
block3c_expand_activation (Acti (None, None, None, 3 0 block3c_expand_bn[0][0]
__________________________________________________________________________________________________
block3c_dwconv (DepthwiseConv2D (None, None, None, 3 9600 block3c_expand_activation[0][0]
__________________________________________________________________________________________________
block3c_bn (BatchNormalization) (None, None, None, 3 1536 block3c_dwconv[0][0]
__________________________________________________________________________________________________
block3c_activation (Activation) (None, None, None, 3 0 block3c_bn[0][0]
__________________________________________________________________________________________________
block3c_se_squeeze (GlobalAvera (None, 384) 0 block3c_activation[0][0]
__________________________________________________________________________________________________
block3c_se_reshape (Reshape) (None, 1, 1, 384) 0 block3c_se_squeeze[0][0]
__________________________________________________________________________________________________
block3c_se_reduce (Conv2D) (None, 1, 1, 16) 6160 block3c_se_reshape[0][0]
__________________________________________________________________________________________________
block3c_se_expand (Conv2D) (None, 1, 1, 384) 6528 block3c_se_reduce[0][0]
__________________________________________________________________________________________________
block3c_se_excite (Multiply) (None, None, None, 3 0 block3c_activation[0][0]
block3c_se_expand[0][0]
__________________________________________________________________________________________________
block3c_project_conv (Conv2D) (None, None, None, 6 24576 block3c_se_excite[0][0]
__________________________________________________________________________________________________
block3c_project_bn (BatchNormal (None, None, None, 6 256 block3c_project_conv[0][0]
__________________________________________________________________________________________________
block3c_drop (FixedDropout) (None, None, None, 6 0 block3c_project_bn[0][0]
__________________________________________________________________________________________________
block3c_add (Add) (None, None, None, 6 0 block3c_drop[0][0]
block3b_add[0][0]
__________________________________________________________________________________________________
block3d_expand_conv (Conv2D) (None, None, None, 3 24576 block3c_add[0][0]
__________________________________________________________________________________________________
block3d_expand_bn (BatchNormali (None, None, None, 3 1536 block3d_expand_conv[0][0]
__________________________________________________________________________________________________
block3d_expand_activation (Acti (None, None, None, 3 0 block3d_expand_bn[0][0]
__________________________________________________________________________________________________
block3d_dwconv (DepthwiseConv2D (None, None, None, 3 9600 block3d_expand_activation[0][0]
__________________________________________________________________________________________________
block3d_bn (BatchNormalization) (None, None, None, 3 1536 block3d_dwconv[0][0]
__________________________________________________________________________________________________
block3d_activation (Activation) (None, None, None, 3 0 block3d_bn[0][0]
__________________________________________________________________________________________________
block3d_se_squeeze (GlobalAvera (None, 384) 0 block3d_activation[0][0]
__________________________________________________________________________________________________
block3d_se_reshape (Reshape) (None, 1, 1, 384) 0 block3d_se_squeeze[0][0]
__________________________________________________________________________________________________
block3d_se_reduce (Conv2D) (None, 1, 1, 16) 6160 block3d_se_reshape[0][0]
__________________________________________________________________________________________________
block3d_se_expand (Conv2D) (None, 1, 1, 384) 6528 block3d_se_reduce[0][0]
__________________________________________________________________________________________________
block3d_se_excite (Multiply) (None, None, None, 3 0 block3d_activation[0][0]
block3d_se_expand[0][0]
__________________________________________________________________________________________________
block3d_project_conv (Conv2D) (None, None, None, 6 24576 block3d_se_excite[0][0]
__________________________________________________________________________________________________
block3d_project_bn (BatchNormal (None, None, None, 6 256 block3d_project_conv[0][0]
__________________________________________________________________________________________________
block3d_drop (FixedDropout) (None, None, None, 6 0 block3d_project_bn[0][0]
__________________________________________________________________________________________________
block3d_add (Add) (None, None, None, 6 0 block3d_drop[0][0]
block3c_add[0][0]
__________________________________________________________________________________________________
block3e_expand_conv (Conv2D) (None, None, None, 3 24576 block3d_add[0][0]
__________________________________________________________________________________________________
block3e_expand_bn (BatchNormali (None, None, None, 3 1536 block3e_expand_conv[0][0]
__________________________________________________________________________________________________
block3e_expand_activation (Acti (None, None, None, 3 0 block3e_expand_bn[0][0]
__________________________________________________________________________________________________
block3e_dwconv (DepthwiseConv2D (None, None, None, 3 9600 block3e_expand_activation[0][0]
__________________________________________________________________________________________________
block3e_bn (BatchNormalization) (None, None, None, 3 1536 block3e_dwconv[0][0]
__________________________________________________________________________________________________
block3e_activation (Activation) (None, None, None, 3 0 block3e_bn[0][0]
__________________________________________________________________________________________________
block3e_se_squeeze (GlobalAvera (None, 384) 0 block3e_activation[0][0]
__________________________________________________________________________________________________
block3e_se_reshape (Reshape) (None, 1, 1, 384) 0 block3e_se_squeeze[0][0]
__________________________________________________________________________________________________
block3e_se_reduce (Conv2D) (None, 1, 1, 16) 6160 block3e_se_reshape[0][0]
__________________________________________________________________________________________________
block3e_se_expand (Conv2D) (None, 1, 1, 384) 6528 block3e_se_reduce[0][0]
__________________________________________________________________________________________________
block3e_se_excite (Multiply) (None, None, None, 3 0 block3e_activation[0][0]
block3e_se_expand[0][0]
__________________________________________________________________________________________________
block3e_project_conv (Conv2D) (None, None, None, 6 24576 block3e_se_excite[0][0]
__________________________________________________________________________________________________
block3e_project_bn (BatchNormal (None, None, None, 6 256 block3e_project_conv[0][0]
__________________________________________________________________________________________________
block3e_drop (FixedDropout) (None, None, None, 6 0 block3e_project_bn[0][0]
__________________________________________________________________________________________________
block3e_add (Add) (None, None, None, 6 0 block3e_drop[0][0]
block3d_add[0][0]
__________________________________________________________________________________________________
block4a_expand_conv (Conv2D) (None, None, None, 3 24576 block3e_add[0][0]
__________________________________________________________________________________________________
block4a_expand_bn (BatchNormali (None, None, None, 3 1536 block4a_expand_conv[0][0]
__________________________________________________________________________________________________
block4a_expand_activation (Acti (None, None, None, 3 0 block4a_expand_bn[0][0]
__________________________________________________________________________________________________
block4a_dwconv (DepthwiseConv2D (None, None, None, 3 3456 block4a_expand_activation[0][0]
__________________________________________________________________________________________________
block4a_bn (BatchNormalization) (None, None, None, 3 1536 block4a_dwconv[0][0]
__________________________________________________________________________________________________
block4a_activation (Activation) (None, None, None, 3 0 block4a_bn[0][0]
__________________________________________________________________________________________________
block4a_se_squeeze (GlobalAvera (None, 384) 0 block4a_activation[0][0]
__________________________________________________________________________________________________
block4a_se_reshape (Reshape) (None, 1, 1, 384) 0 block4a_se_squeeze[0][0]
__________________________________________________________________________________________________
block4a_se_reduce (Conv2D) (None, 1, 1, 16) 6160 block4a_se_reshape[0][0]
__________________________________________________________________________________________________
block4a_se_expand (Conv2D) (None, 1, 1, 384) 6528 block4a_se_reduce[0][0]
__________________________________________________________________________________________________
block4a_se_excite (Multiply) (None, None, None, 3 0 block4a_activation[0][0]
block4a_se_expand[0][0]
__________________________________________________________________________________________________
block4a_project_conv (Conv2D) (None, None, None, 1 49152 block4a_se_excite[0][0]
__________________________________________________________________________________________________
block4a_project_bn (BatchNormal (None, None, None, 1 512 block4a_project_conv[0][0]
__________________________________________________________________________________________________
block4b_expand_conv (Conv2D) (None, None, None, 7 98304 block4a_project_bn[0][0]
__________________________________________________________________________________________________
block4b_expand_bn (BatchNormali (None, None, None, 7 3072 block4b_expand_conv[0][0]
__________________________________________________________________________________________________
block4b_expand_activation (Acti (None, None, None, 7 0 block4b_expand_bn[0][0]
__________________________________________________________________________________________________
block4b_dwconv (DepthwiseConv2D (None, None, None, 7 6912 block4b_expand_activation[0][0]
__________________________________________________________________________________________________
block4b_bn (BatchNormalization) (None, None, None, 7 3072 block4b_dwconv[0][0]
__________________________________________________________________________________________________
block4b_activation (Activation) (None, None, None, 7 0 block4b_bn[0][0]
__________________________________________________________________________________________________
block4b_se_squeeze (GlobalAvera (None, 768) 0 block4b_activation[0][0]
__________________________________________________________________________________________________
block4b_se_reshape (Reshape) (None, 1, 1, 768) 0 block4b_se_squeeze[0][0]
__________________________________________________________________________________________________
block4b_se_reduce (Conv2D) (None, 1, 1, 32) 24608 block4b_se_reshape[0][0]
__________________________________________________________________________________________________
block4b_se_expand (Conv2D) (None, 1, 1, 768) 25344 block4b_se_reduce[0][0]
__________________________________________________________________________________________________
block4b_se_excite (Multiply) (None, None, None, 7 0 block4b_activation[0][0]
block4b_se_expand[0][0]
__________________________________________________________________________________________________
block4b_project_conv (Conv2D) (None, None, None, 1 98304 block4b_se_excite[0][0]
__________________________________________________________________________________________________
block4b_project_bn (BatchNormal (None, None, None, 1 512 block4b_project_conv[0][0]
__________________________________________________________________________________________________
block4b_drop (FixedDropout) (None, None, None, 1 0 block4b_project_bn[0][0]
__________________________________________________________________________________________________
block4b_add (Add) (None, None, None, 1 0 block4b_drop[0][0]
block4a_project_bn[0][0]
__________________________________________________________________________________________________
block4c_expand_conv (Conv2D) (None, None, None, 7 98304 block4b_add[0][0]
__________________________________________________________________________________________________
block4c_expand_bn (BatchNormali (None, None, None, 7 3072 block4c_expand_conv[0][0]
__________________________________________________________________________________________________
block4c_expand_activation (Acti (None, None, None, 7 0 block4c_expand_bn[0][0]
__________________________________________________________________________________________________
block4c_dwconv (DepthwiseConv2D (None, None, None, 7 6912 block4c_expand_activation[0][0]
__________________________________________________________________________________________________
block4c_bn (BatchNormalization) (None, None, None, 7 3072 block4c_dwconv[0][0]
__________________________________________________________________________________________________
block4c_activation (Activation) (None, None, None, 7 0 block4c_bn[0][0]
__________________________________________________________________________________________________
block4c_se_squeeze (GlobalAvera (None, 768) 0 block4c_activation[0][0]
__________________________________________________________________________________________________
block4c_se_reshape (Reshape) (None, 1, 1, 768) 0 block4c_se_squeeze[0][0]
__________________________________________________________________________________________________
block4c_se_reduce (Conv2D) (None, 1, 1, 32) 24608 block4c_se_reshape[0][0]
__________________________________________________________________________________________________
block4c_se_expand (Conv2D) (None, 1, 1, 768) 25344 block4c_se_reduce[0][0]
__________________________________________________________________________________________________
block4c_se_excite (Multiply) (None, None, None, 7 0 block4c_activation[0][0]
block4c_se_expand[0][0]
__________________________________________________________________________________________________
block4c_project_conv (Conv2D) (None, None, None, 1 98304 block4c_se_excite[0][0]
__________________________________________________________________________________________________
block4c_project_bn (BatchNormal (None, None, None, 1 512 block4c_project_conv[0][0]
__________________________________________________________________________________________________
block4c_drop (FixedDropout) (None, None, None, 1 0 block4c_project_bn[0][0]
__________________________________________________________________________________________________
block4c_add (Add) (None, None, None, 1 0 block4c_drop[0][0]
block4b_add[0][0]
__________________________________________________________________________________________________
block4d_expand_conv (Conv2D) (None, None, None, 7 98304 block4c_add[0][0]
__________________________________________________________________________________________________
block4d_expand_bn (BatchNormali (None, None, None, 7 3072 block4d_expand_conv[0][0]
__________________________________________________________________________________________________
block4d_expand_activation (Acti (None, None, None, 7 0 block4d_expand_bn[0][0]
__________________________________________________________________________________________________
block4d_dwconv (DepthwiseConv2D (None, None, None, 7 6912 block4d_expand_activation[0][0]
__________________________________________________________________________________________________
block4d_bn (BatchNormalization) (None, None, None, 7 3072 block4d_dwconv[0][0]
__________________________________________________________________________________________________
block4d_activation (Activation) (None, None, None, 7 0 block4d_bn[0][0]
__________________________________________________________________________________________________
block4d_se_squeeze (GlobalAvera (None, 768) 0 block4d_activation[0][0]
__________________________________________________________________________________________________
block4d_se_reshape (Reshape) (None, 1, 1, 768) 0 block4d_se_squeeze[0][0]
__________________________________________________________________________________________________
block4d_se_reduce (Conv2D) (None, 1, 1, 32) 24608 block4d_se_reshape[0][0]
__________________________________________________________________________________________________
block4d_se_expand (Conv2D) (None, 1, 1, 768) 25344 block4d_se_reduce[0][0]
__________________________________________________________________________________________________
block4d_se_excite (Multiply) (None, None, None, 7 0 block4d_activation[0][0]
block4d_se_expand[0][0]
__________________________________________________________________________________________________
block4d_project_conv (Conv2D) (None, None, None, 1 98304 block4d_se_excite[0][0]
__________________________________________________________________________________________________
block4d_project_bn (BatchNormal (None, None, None, 1 512 block4d_project_conv[0][0]
__________________________________________________________________________________________________
block4d_drop (FixedDropout) (None, None, None, 1 0 block4d_project_bn[0][0]
__________________________________________________________________________________________________
block4d_add (Add) (None, None, None, 1 0 block4d_drop[0][0]
block4c_add[0][0]
__________________________________________________________________________________________________
block4e_expand_conv (Conv2D) (None, None, None, 7 98304 block4d_add[0][0]
__________________________________________________________________________________________________
block4e_expand_bn (BatchNormali (None, None, None, 7 3072 block4e_expand_conv[0][0]
__________________________________________________________________________________________________
block4e_expand_activation (Acti (None, None, None, 7 0 block4e_expand_bn[0][0]
__________________________________________________________________________________________________
block4e_dwconv (DepthwiseConv2D (None, None, None, 7 6912 block4e_expand_activation[0][0]
__________________________________________________________________________________________________
block4e_bn (BatchNormalization) (None, None, None, 7 3072 block4e_dwconv[0][0]
__________________________________________________________________________________________________
block4e_activation (Activation) (None, None, None, 7 0 block4e_bn[0][0]
__________________________________________________________________________________________________
block4e_se_squeeze (GlobalAvera (None, 768) 0 block4e_activation[0][0]
__________________________________________________________________________________________________
block4e_se_reshape (Reshape) (None, 1, 1, 768) 0 block4e_se_squeeze[0][0]
__________________________________________________________________________________________________
block4e_se_reduce (Conv2D) (None, 1, 1, 32) 24608 block4e_se_reshape[0][0]
__________________________________________________________________________________________________
block4e_se_expand (Conv2D) (None, 1, 1, 768) 25344 block4e_se_reduce[0][0]
__________________________________________________________________________________________________
block4e_se_excite (Multiply) (None, None, None, 7 0 block4e_activation[0][0]
block4e_se_expand[0][0]
__________________________________________________________________________________________________
block4e_project_conv (Conv2D) (None, None, None, 1 98304 block4e_se_excite[0][0]
__________________________________________________________________________________________________
block4e_project_bn (BatchNormal (None, None, None, 1 512 block4e_project_conv[0][0]
__________________________________________________________________________________________________
block4e_drop (FixedDropout) (None, None, None, 1 0 block4e_project_bn[0][0]
__________________________________________________________________________________________________
block4e_add (Add) (None, None, None, 1 0 block4e_drop[0][0]
block4d_add[0][0]
__________________________________________________________________________________________________
block4f_expand_conv (Conv2D) (None, None, None, 7 98304 block4e_add[0][0]
__________________________________________________________________________________________________
block4f_expand_bn (BatchNormali (None, None, None, 7 3072 block4f_expand_conv[0][0]
__________________________________________________________________________________________________
block4f_expand_activation (Acti (None, None, None, 7 0 block4f_expand_bn[0][0]
__________________________________________________________________________________________________
block4f_dwconv (DepthwiseConv2D (None, None, None, 7 6912 block4f_expand_activation[0][0]
__________________________________________________________________________________________________
block4f_bn (BatchNormalization) (None, None, None, 7 3072 block4f_dwconv[0][0]
__________________________________________________________________________________________________
block4f_activation (Activation) (None, None, None, 7 0 block4f_bn[0][0]
__________________________________________________________________________________________________
block4f_se_squeeze (GlobalAvera (None, 768) 0 block4f_activation[0][0]
__________________________________________________________________________________________________
block4f_se_reshape (Reshape) (None, 1, 1, 768) 0 block4f_se_squeeze[0][0]
__________________________________________________________________________________________________
block4f_se_reduce (Conv2D) (None, 1, 1, 32) 24608 block4f_se_reshape[0][0]
__________________________________________________________________________________________________
block4f_se_expand (Conv2D) (None, 1, 1, 768) 25344 block4f_se_reduce[0][0]
__________________________________________________________________________________________________
block4f_se_excite (Multiply) (None, None, None, 7 0 block4f_activation[0][0]
block4f_se_expand[0][0]
__________________________________________________________________________________________________
block4f_project_conv (Conv2D) (None, None, None, 1 98304 block4f_se_excite[0][0]
__________________________________________________________________________________________________
block4f_project_bn (BatchNormal (None, None, None, 1 512 block4f_project_conv[0][0]
__________________________________________________________________________________________________
block4f_drop (FixedDropout) (None, None, None, 1 0 block4f_project_bn[0][0]
__________________________________________________________________________________________________
block4f_add (Add) (None, None, None, 1 0 block4f_drop[0][0]
block4e_add[0][0]
__________________________________________________________________________________________________
block4g_expand_conv (Conv2D) (None, None, None, 7 98304 block4f_add[0][0]
__________________________________________________________________________________________________
block4g_expand_bn (BatchNormali (None, None, None, 7 3072 block4g_expand_conv[0][0]
__________________________________________________________________________________________________
block4g_expand_activation (Acti (None, None, None, 7 0 block4g_expand_bn[0][0]
__________________________________________________________________________________________________
block4g_dwconv (DepthwiseConv2D (None, None, None, 7 6912 block4g_expand_activation[0][0]
__________________________________________________________________________________________________
block4g_bn (BatchNormalization) (None, None, None, 7 3072 block4g_dwconv[0][0]
__________________________________________________________________________________________________
block4g_activation (Activation) (None, None, None, 7 0 block4g_bn[0][0]
__________________________________________________________________________________________________
block4g_se_squeeze (GlobalAvera (None, 768) 0 block4g_activation[0][0]
__________________________________________________________________________________________________
block4g_se_reshape (Reshape) (None, 1, 1, 768) 0 block4g_se_squeeze[0][0]
__________________________________________________________________________________________________
block4g_se_reduce (Conv2D) (None, 1, 1, 32) 24608 block4g_se_reshape[0][0]
__________________________________________________________________________________________________
block4g_se_expand (Conv2D) (None, 1, 1, 768) 25344 block4g_se_reduce[0][0]
__________________________________________________________________________________________________
block4g_se_excite (Multiply) (None, None, None, 7 0 block4g_activation[0][0]
block4g_se_expand[0][0]
__________________________________________________________________________________________________
block4g_project_conv (Conv2D) (None, None, None, 1 98304 block4g_se_excite[0][0]
__________________________________________________________________________________________________
block4g_project_bn (BatchNormal (None, None, None, 1 512 block4g_project_conv[0][0]
__________________________________________________________________________________________________
block4g_drop (FixedDropout) (None, None, None, 1 0 block4g_project_bn[0][0]
__________________________________________________________________________________________________
block4g_add (Add) (None, None, None, 1 0 block4g_drop[0][0]
block4f_add[0][0]
__________________________________________________________________________________________________
block5a_expand_conv (Conv2D) (None, None, None, 7 98304 block4g_add[0][0]
__________________________________________________________________________________________________
block5a_expand_bn (BatchNormali (None, None, None, 7 3072 block5a_expand_conv[0][0]
__________________________________________________________________________________________________
block5a_expand_activation (Acti (None, None, None, 7 0 block5a_expand_bn[0][0]
__________________________________________________________________________________________________
block5a_dwconv (DepthwiseConv2D (None, None, None, 7 19200 block5a_expand_activation[0][0]
__________________________________________________________________________________________________
block5a_bn (BatchNormalization) (None, None, None, 7 3072 block5a_dwconv[0][0]
__________________________________________________________________________________________________
block5a_activation (Activation) (None, None, None, 7 0 block5a_bn[0][0]
__________________________________________________________________________________________________
block5a_se_squeeze (GlobalAvera (None, 768) 0 block5a_activation[0][0]
__________________________________________________________________________________________________
block5a_se_reshape (Reshape) (None, 1, 1, 768) 0 block5a_se_squeeze[0][0]
__________________________________________________________________________________________________
block5a_se_reduce (Conv2D) (None, 1, 1, 32) 24608 block5a_se_reshape[0][0]
__________________________________________________________________________________________________
block5a_se_expand (Conv2D) (None, 1, 1, 768) 25344 block5a_se_reduce[0][0]
__________________________________________________________________________________________________
block5a_se_excite (Multiply) (None, None, None, 7 0 block5a_activation[0][0]
block5a_se_expand[0][0]
__________________________________________________________________________________________________
block5a_project_conv (Conv2D) (None, None, None, 1 135168 block5a_se_excite[0][0]
__________________________________________________________________________________________________
block5a_project_bn (BatchNormal (None, None, None, 1 704 block5a_project_conv[0][0]
__________________________________________________________________________________________________
block5b_expand_conv (Conv2D) (None, None, None, 1 185856 block5a_project_bn[0][0]
__________________________________________________________________________________________________
block5b_expand_bn (BatchNormali (None, None, None, 1 4224 block5b_expand_conv[0][0]
__________________________________________________________________________________________________
block5b_expand_activation (Acti (None, None, None, 1 0 block5b_expand_bn[0][0]
__________________________________________________________________________________________________
block5b_dwconv (DepthwiseConv2D (None, None, None, 1 26400 block5b_expand_activation[0][0]
__________________________________________________________________________________________________
block5b_bn (BatchNormalization) (None, None, None, 1 4224 block5b_dwconv[0][0]
__________________________________________________________________________________________________
block5b_activation (Activation) (None, None, None, 1 0 block5b_bn[0][0]
__________________________________________________________________________________________________
block5b_se_squeeze (GlobalAvera (None, 1056) 0 block5b_activation[0][0]
__________________________________________________________________________________________________
block5b_se_reshape (Reshape) (None, 1, 1, 1056) 0 block5b_se_squeeze[0][0]
__________________________________________________________________________________________________
block5b_se_reduce (Conv2D) (None, 1, 1, 44) 46508 block5b_se_reshape[0][0]
__________________________________________________________________________________________________
block5b_se_expand (Conv2D) (None, 1, 1, 1056) 47520 block5b_se_reduce[0][0]
__________________________________________________________________________________________________
block5b_se_excite (Multiply) (None, None, None, 1 0 block5b_activation[0][0]
block5b_se_expand[0][0]
__________________________________________________________________________________________________
block5b_project_conv (Conv2D) (None, None, None, 1 185856 block5b_se_excite[0][0]
__________________________________________________________________________________________________
block5b_project_bn (BatchNormal (None, None, None, 1 704 block5b_project_conv[0][0]
__________________________________________________________________________________________________
block5b_drop (FixedDropout) (None, None, None, 1 0 block5b_project_bn[0][0]
__________________________________________________________________________________________________
block5b_add (Add) (None, None, None, 1 0 block5b_drop[0][0]
block5a_project_bn[0][0]
__________________________________________________________________________________________________
block5c_expand_conv (Conv2D) (None, None, None, 1 185856 block5b_add[0][0]
__________________________________________________________________________________________________
block5c_expand_bn (BatchNormali (None, None, None, 1 4224 block5c_expand_conv[0][0]
__________________________________________________________________________________________________
block5c_expand_activation (Acti (None, None, None, 1 0 block5c_expand_bn[0][0]
__________________________________________________________________________________________________
block5c_dwconv (DepthwiseConv2D (None, None, None, 1 26400 block5c_expand_activation[0][0]
__________________________________________________________________________________________________
block5c_bn (BatchNormalization) (None, None, None, 1 4224 block5c_dwconv[0][0]
__________________________________________________________________________________________________
block5c_activation (Activation) (None, None, None, 1 0 block5c_bn[0][0]
__________________________________________________________________________________________________
block5c_se_squeeze (GlobalAvera (None, 1056) 0 block5c_activation[0][0]
__________________________________________________________________________________________________
block5c_se_reshape (Reshape) (None, 1, 1, 1056) 0 block5c_se_squeeze[0][0]
__________________________________________________________________________________________________
block5c_se_reduce (Conv2D) (None, 1, 1, 44) 46508 block5c_se_reshape[0][0]
__________________________________________________________________________________________________
block5c_se_expand (Conv2D) (None, 1, 1, 1056) 47520 block5c_se_reduce[0][0]
__________________________________________________________________________________________________
block5c_se_excite (Multiply) (None, None, None, 1 0 block5c_activation[0][0]
block5c_se_expand[0][0]
__________________________________________________________________________________________________
block5c_project_conv (Conv2D) (None, None, None, 1 185856 block5c_se_excite[0][0]
__________________________________________________________________________________________________
block5c_project_bn (BatchNormal (None, None, None, 1 704 block5c_project_conv[0][0]
__________________________________________________________________________________________________
block5c_drop (FixedDropout) (None, None, None, 1 0 block5c_project_bn[0][0]
__________________________________________________________________________________________________
block5c_add (Add) (None, None, None, 1 0 block5c_drop[0][0]
block5b_add[0][0]
__________________________________________________________________________________________________
block5d_expand_conv (Conv2D) (None, None, None, 1 185856 block5c_add[0][0]
__________________________________________________________________________________________________
block5d_expand_bn (BatchNormali (None, None, None, 1 4224 block5d_expand_conv[0][0]
__________________________________________________________________________________________________
block5d_expand_activation (Acti (None, None, None, 1 0 block5d_expand_bn[0][0]
__________________________________________________________________________________________________
block5d_dwconv (DepthwiseConv2D (None, None, None, 1 26400 block5d_expand_activation[0][0]
__________________________________________________________________________________________________
block5d_bn (BatchNormalization) (None, None, None, 1 4224 block5d_dwconv[0][0]
__________________________________________________________________________________________________
block5d_activation (Activation) (None, None, None, 1 0 block5d_bn[0][0]
__________________________________________________________________________________________________
block5d_se_squeeze (GlobalAvera (None, 1056) 0 block5d_activation[0][0]
__________________________________________________________________________________________________
block5d_se_reshape (Reshape) (None, 1, 1, 1056) 0 block5d_se_squeeze[0][0]
__________________________________________________________________________________________________
block5d_se_reduce (Conv2D) (None, 1, 1, 44) 46508 block5d_se_reshape[0][0]
__________________________________________________________________________________________________
block5d_se_expand (Conv2D) (None, 1, 1, 1056) 47520 block5d_se_reduce[0][0]
__________________________________________________________________________________________________
block5d_se_excite (Multiply) (None, None, None, 1 0 block5d_activation[0][0]
block5d_se_expand[0][0]
__________________________________________________________________________________________________
block5d_project_conv (Conv2D) (None, None, None, 1 185856 block5d_se_excite[0][0]
__________________________________________________________________________________________________
block5d_project_bn (BatchNormal (None, None, None, 1 704 block5d_project_conv[0][0]
__________________________________________________________________________________________________
block5d_drop (FixedDropout) (None, None, None, 1 0 block5d_project_bn[0][0]
__________________________________________________________________________________________________
block5d_add (Add) (None, None, None, 1 0 block5d_drop[0][0]
block5c_add[0][0]
__________________________________________________________________________________________________
block5e_expand_conv (Conv2D) (None, None, None, 1 185856 block5d_add[0][0]
__________________________________________________________________________________________________
block5e_expand_bn (BatchNormali (None, None, None, 1 4224 block5e_expand_conv[0][0]
__________________________________________________________________________________________________
block5e_expand_activation (Acti (None, None, None, 1 0 block5e_expand_bn[0][0]
__________________________________________________________________________________________________
block5e_dwconv (DepthwiseConv2D (None, None, None, 1 26400 block5e_expand_activation[0][0]
__________________________________________________________________________________________________
block5e_bn (BatchNormalization) (None, None, None, 1 4224 block5e_dwconv[0][0]
__________________________________________________________________________________________________
block5e_activation (Activation) (None, None, None, 1 0 block5e_bn[0][0]
__________________________________________________________________________________________________
block5e_se_squeeze (GlobalAvera (None, 1056) 0 block5e_activation[0][0]
__________________________________________________________________________________________________
block5e_se_reshape (Reshape) (None, 1, 1, 1056) 0 block5e_se_squeeze[0][0]
__________________________________________________________________________________________________
block5e_se_reduce (Conv2D) (None, 1, 1, 44) 46508 block5e_se_reshape[0][0]
__________________________________________________________________________________________________
block5e_se_expand (Conv2D) (None, 1, 1, 1056) 47520 block5e_se_reduce[0][0]
__________________________________________________________________________________________________
block5e_se_excite (Multiply) (None, None, None, 1 0 block5e_activation[0][0]
block5e_se_expand[0][0]
__________________________________________________________________________________________________
block5e_project_conv (Conv2D) (None, None, None, 1 185856 block5e_se_excite[0][0]
__________________________________________________________________________________________________
block5e_project_bn (BatchNormal (None, None, None, 1 704 block5e_project_conv[0][0]
__________________________________________________________________________________________________
block5e_drop (FixedDropout) (None, None, None, 1 0 block5e_project_bn[0][0]
__________________________________________________________________________________________________
block5e_add (Add) (None, None, None, 1 0 block5e_drop[0][0]
block5d_add[0][0]
__________________________________________________________________________________________________
block5f_expand_conv (Conv2D) (None, None, None, 1 185856 block5e_add[0][0]
__________________________________________________________________________________________________
block5f_expand_bn (BatchNormali (None, None, None, 1 4224 block5f_expand_conv[0][0]
__________________________________________________________________________________________________
block5f_expand_activation (Acti (None, None, None, 1 0 block5f_expand_bn[0][0]
__________________________________________________________________________________________________
block5f_dwconv (DepthwiseConv2D (None, None, None, 1 26400 block5f_expand_activation[0][0]
__________________________________________________________________________________________________
block5f_bn (BatchNormalization) (None, None, None, 1 4224 block5f_dwconv[0][0]
__________________________________________________________________________________________________
block5f_activation (Activation) (None, None, None, 1 0 block5f_bn[0][0]
__________________________________________________________________________________________________
block5f_se_squeeze (GlobalAvera (None, 1056) 0 block5f_activation[0][0]
__________________________________________________________________________________________________
block5f_se_reshape (Reshape) (None, 1, 1, 1056) 0 block5f_se_squeeze[0][0]
__________________________________________________________________________________________________
block5f_se_reduce (Conv2D) (None, 1, 1, 44) 46508 block5f_se_reshape[0][0]
__________________________________________________________________________________________________
block5f_se_expand (Conv2D) (None, 1, 1, 1056) 47520 block5f_se_reduce[0][0]
__________________________________________________________________________________________________
block5f_se_excite (Multiply) (None, None, None, 1 0 block5f_activation[0][0]
block5f_se_expand[0][0]
__________________________________________________________________________________________________
block5f_project_conv (Conv2D) (None, None, None, 1 185856 block5f_se_excite[0][0]
__________________________________________________________________________________________________
block5f_project_bn (BatchNormal (None, None, None, 1 704 block5f_project_conv[0][0]
__________________________________________________________________________________________________
block5f_drop (FixedDropout) (None, None, None, 1 0 block5f_project_bn[0][0]
__________________________________________________________________________________________________
block5f_add (Add) (None, None, None, 1 0 block5f_drop[0][0]
block5e_add[0][0]
__________________________________________________________________________________________________
block5g_expand_conv (Conv2D) (None, None, None, 1 185856 block5f_add[0][0]
__________________________________________________________________________________________________
block5g_expand_bn (BatchNormali (None, None, None, 1 4224 block5g_expand_conv[0][0]
__________________________________________________________________________________________________
block5g_expand_activation (Acti (None, None, None, 1 0 block5g_expand_bn[0][0]
__________________________________________________________________________________________________
block5g_dwconv (DepthwiseConv2D (None, None, None, 1 26400 block5g_expand_activation[0][0]
__________________________________________________________________________________________________
block5g_bn (BatchNormalization) (None, None, None, 1 4224 block5g_dwconv[0][0]
__________________________________________________________________________________________________
block5g_activation (Activation) (None, None, None, 1 0 block5g_bn[0][0]
__________________________________________________________________________________________________
block5g_se_squeeze (GlobalAvera (None, 1056) 0 block5g_activation[0][0]
__________________________________________________________________________________________________
block5g_se_reshape (Reshape) (None, 1, 1, 1056) 0 block5g_se_squeeze[0][0]
__________________________________________________________________________________________________
block5g_se_reduce (Conv2D) (None, 1, 1, 44) 46508 block5g_se_reshape[0][0]
__________________________________________________________________________________________________
block5g_se_expand (Conv2D) (None, 1, 1, 1056) 47520 block5g_se_reduce[0][0]
__________________________________________________________________________________________________
block5g_se_excite (Multiply) (None, None, None, 1 0 block5g_activation[0][0]
block5g_se_expand[0][0]
__________________________________________________________________________________________________
block5g_project_conv (Conv2D) (None, None, None, 1 185856 block5g_se_excite[0][0]
__________________________________________________________________________________________________
block5g_project_bn (BatchNormal (None, None, None, 1 704 block5g_project_conv[0][0]
__________________________________________________________________________________________________
block5g_drop (FixedDropout) (None, None, None, 1 0 block5g_project_bn[0][0]
__________________________________________________________________________________________________
block5g_add (Add) (None, None, None, 1 0 block5g_drop[0][0]
block5f_add[0][0]
__________________________________________________________________________________________________
block6a_expand_conv (Conv2D) (None, None, None, 1 185856 block5g_add[0][0]
__________________________________________________________________________________________________
block6a_expand_bn (BatchNormali (None, None, None, 1 4224 block6a_expand_conv[0][0]
__________________________________________________________________________________________________
block6a_expand_activation (Acti (None, None, None, 1 0 block6a_expand_bn[0][0]
__________________________________________________________________________________________________
block6a_dwconv (DepthwiseConv2D (None, None, None, 1 26400 block6a_expand_activation[0][0]
__________________________________________________________________________________________________
block6a_bn (BatchNormalization) (None, None, None, 1 4224 block6a_dwconv[0][0]
__________________________________________________________________________________________________
block6a_activation (Activation) (None, None, None, 1 0 block6a_bn[0][0]
__________________________________________________________________________________________________
block6a_se_squeeze (GlobalAvera (None, 1056) 0 block6a_activation[0][0]
__________________________________________________________________________________________________
block6a_se_reshape (Reshape) (None, 1, 1, 1056) 0 block6a_se_squeeze[0][0]
__________________________________________________________________________________________________
block6a_se_reduce (Conv2D) (None, 1, 1, 44) 46508 block6a_se_reshape[0][0]
__________________________________________________________________________________________________
block6a_se_expand (Conv2D) (None, 1, 1, 1056) 47520 block6a_se_reduce[0][0]
__________________________________________________________________________________________________
block6a_se_excite (Multiply) (None, None, None, 1 0 block6a_activation[0][0]
block6a_se_expand[0][0]
__________________________________________________________________________________________________
block6a_project_conv (Conv2D) (None, None, None, 3 321024 block6a_se_excite[0][0]
__________________________________________________________________________________________________
block6a_project_bn (BatchNormal (None, None, None, 3 1216 block6a_project_conv[0][0]
__________________________________________________________________________________________________
block6b_expand_conv (Conv2D) (None, None, None, 1 554496 block6a_project_bn[0][0]
__________________________________________________________________________________________________
block6b_expand_bn (BatchNormali (None, None, None, 1 7296 block6b_expand_conv[0][0]
__________________________________________________________________________________________________
block6b_expand_activation (Acti (None, None, None, 1 0 block6b_expand_bn[0][0]
__________________________________________________________________________________________________
block6b_dwconv (DepthwiseConv2D (None, None, None, 1 45600 block6b_expand_activation[0][0]
__________________________________________________________________________________________________
block6b_bn (BatchNormalization) (None, None, None, 1 7296 block6b_dwconv[0][0]
__________________________________________________________________________________________________
block6b_activation (Activation) (None, None, None, 1 0 block6b_bn[0][0]
__________________________________________________________________________________________________
block6b_se_squeeze (GlobalAvera (None, 1824) 0 block6b_activation[0][0]
__________________________________________________________________________________________________
block6b_se_reshape (Reshape) (None, 1, 1, 1824) 0 block6b_se_squeeze[0][0]
__________________________________________________________________________________________________
block6b_se_reduce (Conv2D) (None, 1, 1, 76) 138700 block6b_se_reshape[0][0]
__________________________________________________________________________________________________
block6b_se_expand (Conv2D) (None, 1, 1, 1824) 140448 block6b_se_reduce[0][0]
__________________________________________________________________________________________________
block6b_se_excite (Multiply) (None, None, None, 1 0 block6b_activation[0][0]
block6b_se_expand[0][0]
__________________________________________________________________________________________________
block6b_project_conv (Conv2D) (None, None, None, 3 554496 block6b_se_excite[0][0]
__________________________________________________________________________________________________
block6b_project_bn (BatchNormal (None, None, None, 3 1216 block6b_project_conv[0][0]
__________________________________________________________________________________________________
block6b_drop (FixedDropout) (None, None, None, 3 0 block6b_project_bn[0][0]
__________________________________________________________________________________________________
block6b_add (Add) (None, None, None, 3 0 block6b_drop[0][0]
block6a_project_bn[0][0]
__________________________________________________________________________________________________
block6c_expand_conv (Conv2D) (None, None, None, 1 554496 block6b_add[0][0]
__________________________________________________________________________________________________
block6c_expand_bn (BatchNormali (None, None, None, 1 7296 block6c_expand_conv[0][0]
__________________________________________________________________________________________________
block6c_expand_activation (Acti (None, None, None, 1 0 block6c_expand_bn[0][0]
__________________________________________________________________________________________________
block6c_dwconv (DepthwiseConv2D (None, None, None, 1 45600 block6c_expand_activation[0][0]
__________________________________________________________________________________________________
block6c_bn (BatchNormalization) (None, None, None, 1 7296 block6c_dwconv[0][0]
__________________________________________________________________________________________________
block6c_activation (Activation) (None, None, None, 1 0 block6c_bn[0][0]
__________________________________________________________________________________________________
block6c_se_squeeze (GlobalAvera (None, 1824) 0 block6c_activation[0][0]
__________________________________________________________________________________________________
block6c_se_reshape (Reshape) (None, 1, 1, 1824) 0 block6c_se_squeeze[0][0]
__________________________________________________________________________________________________
block6c_se_reduce (Conv2D) (None, 1, 1, 76) 138700 block6c_se_reshape[0][0]
__________________________________________________________________________________________________
block6c_se_expand (Conv2D) (None, 1, 1, 1824) 140448 block6c_se_reduce[0][0]
__________________________________________________________________________________________________
block6c_se_excite (Multiply) (None, None, None, 1 0 block6c_activation[0][0]
block6c_se_expand[0][0]
__________________________________________________________________________________________________
block6c_project_conv (Conv2D) (None, None, None, 3 554496 block6c_se_excite[0][0]
__________________________________________________________________________________________________
block6c_project_bn (BatchNormal (None, None, None, 3 1216 block6c_project_conv[0][0]
__________________________________________________________________________________________________
block6c_drop (FixedDropout) (None, None, None, 3 0 block6c_project_bn[0][0]
__________________________________________________________________________________________________
block6c_add (Add) (None, None, None, 3 0 block6c_drop[0][0]
block6b_add[0][0]
__________________________________________________________________________________________________
block6d_expand_conv (Conv2D) (None, None, None, 1 554496 block6c_add[0][0]
__________________________________________________________________________________________________
block6d_expand_bn (BatchNormali (None, None, None, 1 7296 block6d_expand_conv[0][0]
__________________________________________________________________________________________________
block6d_expand_activation (Acti (None, None, None, 1 0 block6d_expand_bn[0][0]
__________________________________________________________________________________________________
block6d_dwconv (DepthwiseConv2D (None, None, None, 1 45600 block6d_expand_activation[0][0]
__________________________________________________________________________________________________
block6d_bn (BatchNormalization) (None, None, None, 1 7296 block6d_dwconv[0][0]
__________________________________________________________________________________________________
block6d_activation (Activation) (None, None, None, 1 0 block6d_bn[0][0]
__________________________________________________________________________________________________
block6d_se_squeeze (GlobalAvera (None, 1824) 0 block6d_activation[0][0]
__________________________________________________________________________________________________
block6d_se_reshape (Reshape) (None, 1, 1, 1824) 0 block6d_se_squeeze[0][0]
__________________________________________________________________________________________________
block6d_se_reduce (Conv2D) (None, 1, 1, 76) 138700 block6d_se_reshape[0][0]
__________________________________________________________________________________________________
block6d_se_expand (Conv2D) (None, 1, 1, 1824) 140448 block6d_se_reduce[0][0]
__________________________________________________________________________________________________
block6d_se_excite (Multiply) (None, None, None, 1 0 block6d_activation[0][0]
block6d_se_expand[0][0]
__________________________________________________________________________________________________
block6d_project_conv (Conv2D) (None, None, None, 3 554496 block6d_se_excite[0][0]
__________________________________________________________________________________________________
block6d_project_bn (BatchNormal (None, None, None, 3 1216 block6d_project_conv[0][0]
__________________________________________________________________________________________________
block6d_drop (FixedDropout) (None, None, None, 3 0 block6d_project_bn[0][0]
__________________________________________________________________________________________________
block6d_add (Add) (None, None, None, 3 0 block6d_drop[0][0]
block6c_add[0][0]
__________________________________________________________________________________________________
block6e_expand_conv (Conv2D) (None, None, None, 1 554496 block6d_add[0][0]
__________________________________________________________________________________________________
block6e_expand_bn (BatchNormali (None, None, None, 1 7296 block6e_expand_conv[0][0]
__________________________________________________________________________________________________
block6e_expand_activation (Acti (None, None, None, 1 0 block6e_expand_bn[0][0]
__________________________________________________________________________________________________
block6e_dwconv (DepthwiseConv2D (None, None, None, 1 45600 block6e_expand_activation[0][0]
__________________________________________________________________________________________________
block6e_bn (BatchNormalization) (None, None, None, 1 7296 block6e_dwconv[0][0]
__________________________________________________________________________________________________
block6e_activation (Activation) (None, None, None, 1 0 block6e_bn[0][0]
__________________________________________________________________________________________________
block6e_se_squeeze (GlobalAvera (None, 1824) 0 block6e_activation[0][0]
__________________________________________________________________________________________________
block6e_se_reshape (Reshape) (None, 1, 1, 1824) 0 block6e_se_squeeze[0][0]
__________________________________________________________________________________________________
block6e_se_reduce (Conv2D) (None, 1, 1, 76) 138700 block6e_se_reshape[0][0]
__________________________________________________________________________________________________
block6e_se_expand (Conv2D) (None, 1, 1, 1824) 140448 block6e_se_reduce[0][0]
__________________________________________________________________________________________________
block6e_se_excite (Multiply) (None, None, None, 1 0 block6e_activation[0][0]
block6e_se_expand[0][0]
__________________________________________________________________________________________________
block6e_project_conv (Conv2D) (None, None, None, 3 554496 block6e_se_excite[0][0]
__________________________________________________________________________________________________
block6e_project_bn (BatchNormal (None, None, None, 3 1216 block6e_project_conv[0][0]
__________________________________________________________________________________________________
block6e_drop (FixedDropout) (None, None, None, 3 0 block6e_project_bn[0][0]
__________________________________________________________________________________________________
block6e_add (Add) (None, None, None, 3 0 block6e_drop[0][0]
block6d_add[0][0]
__________________________________________________________________________________________________
block6f_expand_conv (Conv2D) (None, None, None, 1 554496 block6e_add[0][0]
__________________________________________________________________________________________________
block6f_expand_bn (BatchNormali (None, None, None, 1 7296 block6f_expand_conv[0][0]
__________________________________________________________________________________________________
block6f_expand_activation (Acti (None, None, None, 1 0 block6f_expand_bn[0][0]
__________________________________________________________________________________________________
block6f_dwconv (DepthwiseConv2D (None, None, None, 1 45600 block6f_expand_activation[0][0]
__________________________________________________________________________________________________
block6f_bn (BatchNormalization) (None, None, None, 1 7296 block6f_dwconv[0][0]
__________________________________________________________________________________________________
block6f_activation (Activation) (None, None, None, 1 0 block6f_bn[0][0]
__________________________________________________________________________________________________
block6f_se_squeeze (GlobalAvera (None, 1824) 0 block6f_activation[0][0]
__________________________________________________________________________________________________
block6f_se_reshape (Reshape) (None, 1, 1, 1824) 0 block6f_se_squeeze[0][0]
__________________________________________________________________________________________________
block6f_se_reduce (Conv2D) (None, 1, 1, 76) 138700 block6f_se_reshape[0][0]
__________________________________________________________________________________________________
block6f_se_expand (Conv2D) (None, 1, 1, 1824) 140448 block6f_se_reduce[0][0]
__________________________________________________________________________________________________
block6f_se_excite (Multiply) (None, None, None, 1 0 block6f_activation[0][0]
block6f_se_expand[0][0]
__________________________________________________________________________________________________
block6f_project_conv (Conv2D) (None, None, None, 3 554496 block6f_se_excite[0][0]
__________________________________________________________________________________________________
block6f_project_bn (BatchNormal (None, None, None, 3 1216 block6f_project_conv[0][0]
__________________________________________________________________________________________________
block6f_drop (FixedDropout) (None, None, None, 3 0 block6f_project_bn[0][0]
__________________________________________________________________________________________________
block6f_add (Add) (None, None, None, 3 0 block6f_drop[0][0]
block6e_add[0][0]
__________________________________________________________________________________________________
block6g_expand_conv (Conv2D) (None, None, None, 1 554496 block6f_add[0][0]
__________________________________________________________________________________________________
block6g_expand_bn (BatchNormali (None, None, None, 1 7296 block6g_expand_conv[0][0]
__________________________________________________________________________________________________
block6g_expand_activation (Acti (None, None, None, 1 0 block6g_expand_bn[0][0]
__________________________________________________________________________________________________
block6g_dwconv (DepthwiseConv2D (None, None, None, 1 45600 block6g_expand_activation[0][0]
__________________________________________________________________________________________________
block6g_bn (BatchNormalization) (None, None, None, 1 7296 block6g_dwconv[0][0]
__________________________________________________________________________________________________
block6g_activation (Activation) (None, None, None, 1 0 block6g_bn[0][0]
__________________________________________________________________________________________________
block6g_se_squeeze (GlobalAvera (None, 1824) 0 block6g_activation[0][0]
__________________________________________________________________________________________________
block6g_se_reshape (Reshape) (None, 1, 1, 1824) 0 block6g_se_squeeze[0][0]
__________________________________________________________________________________________________
block6g_se_reduce (Conv2D) (None, 1, 1, 76) 138700 block6g_se_reshape[0][0]
__________________________________________________________________________________________________
block6g_se_expand (Conv2D) (None, 1, 1, 1824) 140448 block6g_se_reduce[0][0]
__________________________________________________________________________________________________
block6g_se_excite (Multiply) (None, None, None, 1 0 block6g_activation[0][0]
block6g_se_expand[0][0]
__________________________________________________________________________________________________
block6g_project_conv (Conv2D) (None, None, None, 3 554496 block6g_se_excite[0][0]
__________________________________________________________________________________________________
block6g_project_bn (BatchNormal (None, None, None, 3 1216 block6g_project_conv[0][0]
__________________________________________________________________________________________________
block6g_drop (FixedDropout) (None, None, None, 3 0 block6g_project_bn[0][0]
__________________________________________________________________________________________________
block6g_add (Add) (None, None, None, 3 0 block6g_drop[0][0]
block6f_add[0][0]
__________________________________________________________________________________________________
block6h_expand_conv (Conv2D) (None, None, None, 1 554496 block6g_add[0][0]
__________________________________________________________________________________________________
block6h_expand_bn (BatchNormali (None, None, None, 1 7296 block6h_expand_conv[0][0]
__________________________________________________________________________________________________
block6h_expand_activation (Acti (None, None, None, 1 0 block6h_expand_bn[0][0]
__________________________________________________________________________________________________
block6h_dwconv (DepthwiseConv2D (None, None, None, 1 45600 block6h_expand_activation[0][0]
__________________________________________________________________________________________________
block6h_bn (BatchNormalization) (None, None, None, 1 7296 block6h_dwconv[0][0]
__________________________________________________________________________________________________
block6h_activation (Activation) (None, None, None, 1 0 block6h_bn[0][0]
__________________________________________________________________________________________________
block6h_se_squeeze (GlobalAvera (None, 1824) 0 block6h_activation[0][0]
__________________________________________________________________________________________________
block6h_se_reshape (Reshape) (None, 1, 1, 1824) 0 block6h_se_squeeze[0][0]
__________________________________________________________________________________________________
block6h_se_reduce (Conv2D) (None, 1, 1, 76) 138700 block6h_se_reshape[0][0]
__________________________________________________________________________________________________
block6h_se_expand (Conv2D) (None, 1, 1, 1824) 140448 block6h_se_reduce[0][0]
__________________________________________________________________________________________________
block6h_se_excite (Multiply) (None, None, None, 1 0 block6h_activation[0][0]
block6h_se_expand[0][0]
__________________________________________________________________________________________________
block6h_project_conv (Conv2D) (None, None, None, 3 554496 block6h_se_excite[0][0]
__________________________________________________________________________________________________
block6h_project_bn (BatchNormal (None, None, None, 3 1216 block6h_project_conv[0][0]
__________________________________________________________________________________________________
block6h_drop (FixedDropout) (None, None, None, 3 0 block6h_project_bn[0][0]
__________________________________________________________________________________________________
block6h_add (Add) (None, None, None, 3 0 block6h_drop[0][0]
block6g_add[0][0]
__________________________________________________________________________________________________
block6i_expand_conv (Conv2D) (None, None, None, 1 554496 block6h_add[0][0]
__________________________________________________________________________________________________
block6i_expand_bn (BatchNormali (None, None, None, 1 7296 block6i_expand_conv[0][0]
__________________________________________________________________________________________________
block6i_expand_activation (Acti (None, None, None, 1 0 block6i_expand_bn[0][0]
__________________________________________________________________________________________________
block6i_dwconv (DepthwiseConv2D (None, None, None, 1 45600 block6i_expand_activation[0][0]
__________________________________________________________________________________________________
block6i_bn (BatchNormalization) (None, None, None, 1 7296 block6i_dwconv[0][0]
__________________________________________________________________________________________________
block6i_activation (Activation) (None, None, None, 1 0 block6i_bn[0][0]
__________________________________________________________________________________________________
block6i_se_squeeze (GlobalAvera (None, 1824) 0 block6i_activation[0][0]
__________________________________________________________________________________________________
block6i_se_reshape (Reshape) (None, 1, 1, 1824) 0 block6i_se_squeeze[0][0]
__________________________________________________________________________________________________
block6i_se_reduce (Conv2D) (None, 1, 1, 76) 138700 block6i_se_reshape[0][0]
__________________________________________________________________________________________________
block6i_se_expand (Conv2D) (None, 1, 1, 1824) 140448 block6i_se_reduce[0][0]
__________________________________________________________________________________________________
block6i_se_excite (Multiply) (None, None, None, 1 0 block6i_activation[0][0]
block6i_se_expand[0][0]
__________________________________________________________________________________________________
block6i_project_conv (Conv2D) (None, None, None, 3 554496 block6i_se_excite[0][0]
__________________________________________________________________________________________________
block6i_project_bn (BatchNormal (None, None, None, 3 1216 block6i_project_conv[0][0]
__________________________________________________________________________________________________
block6i_drop (FixedDropout) (None, None, None, 3 0 block6i_project_bn[0][0]
__________________________________________________________________________________________________
block6i_add (Add) (None, None, None, 3 0 block6i_drop[0][0]
block6h_add[0][0]
__________________________________________________________________________________________________
block7a_expand_conv (Conv2D) (None, None, None, 1 554496 block6i_add[0][0]
__________________________________________________________________________________________________
block7a_expand_bn (BatchNormali (None, None, None, 1 7296 block7a_expand_conv[0][0]
__________________________________________________________________________________________________
block7a_expand_activation (Acti (None, None, None, 1 0 block7a_expand_bn[0][0]
__________________________________________________________________________________________________
block7a_dwconv (DepthwiseConv2D (None, None, None, 1 16416 block7a_expand_activation[0][0]
__________________________________________________________________________________________________
block7a_bn (BatchNormalization) (None, None, None, 1 7296 block7a_dwconv[0][0]
__________________________________________________________________________________________________
block7a_activation (Activation) (None, None, None, 1 0 block7a_bn[0][0]
__________________________________________________________________________________________________
block7a_se_squeeze (GlobalAvera (None, 1824) 0 block7a_activation[0][0]
__________________________________________________________________________________________________
block7a_se_reshape (Reshape) (None, 1, 1, 1824) 0 block7a_se_squeeze[0][0]
__________________________________________________________________________________________________
block7a_se_reduce (Conv2D) (None, 1, 1, 76) 138700 block7a_se_reshape[0][0]
__________________________________________________________________________________________________
block7a_se_expand (Conv2D) (None, 1, 1, 1824) 140448 block7a_se_reduce[0][0]
__________________________________________________________________________________________________
block7a_se_excite (Multiply) (None, None, None, 1 0 block7a_activation[0][0]
block7a_se_expand[0][0]
__________________________________________________________________________________________________
block7a_project_conv (Conv2D) (None, None, None, 5 933888 block7a_se_excite[0][0]
__________________________________________________________________________________________________
block7a_project_bn (BatchNormal (None, None, None, 5 2048 block7a_project_conv[0][0]
__________________________________________________________________________________________________
block7b_expand_conv (Conv2D) (None, None, None, 3 1572864 block7a_project_bn[0][0]
__________________________________________________________________________________________________
block7b_expand_bn (BatchNormali (None, None, None, 3 12288 block7b_expand_conv[0][0]
__________________________________________________________________________________________________
block7b_expand_activation (Acti (None, None, None, 3 0 block7b_expand_bn[0][0]
__________________________________________________________________________________________________
block7b_dwconv (DepthwiseConv2D (None, None, None, 3 27648 block7b_expand_activation[0][0]
__________________________________________________________________________________________________
block7b_bn (BatchNormalization) (None, None, None, 3 12288 block7b_dwconv[0][0]
__________________________________________________________________________________________________
block7b_activation (Activation) (None, None, None, 3 0 block7b_bn[0][0]
__________________________________________________________________________________________________
block7b_se_squeeze (GlobalAvera (None, 3072) 0 block7b_activation[0][0]
__________________________________________________________________________________________________
block7b_se_reshape (Reshape) (None, 1, 1, 3072) 0 block7b_se_squeeze[0][0]
__________________________________________________________________________________________________
block7b_se_reduce (Conv2D) (None, 1, 1, 128) 393344 block7b_se_reshape[0][0]
__________________________________________________________________________________________________
block7b_se_expand (Conv2D) (None, 1, 1, 3072) 396288 block7b_se_reduce[0][0]
__________________________________________________________________________________________________
block7b_se_excite (Multiply) (None, None, None, 3 0 block7b_activation[0][0]
block7b_se_expand[0][0]
__________________________________________________________________________________________________
block7b_project_conv (Conv2D) (None, None, None, 5 1572864 block7b_se_excite[0][0]
__________________________________________________________________________________________________
block7b_project_bn (BatchNormal (None, None, None, 5 2048 block7b_project_conv[0][0]
__________________________________________________________________________________________________
block7b_drop (FixedDropout) (None, None, None, 5 0 block7b_project_bn[0][0]
__________________________________________________________________________________________________
block7b_add (Add) (None, None, None, 5 0 block7b_drop[0][0]
block7a_project_bn[0][0]
__________________________________________________________________________________________________
block7c_expand_conv (Conv2D) (None, None, None, 3 1572864 block7b_add[0][0]
__________________________________________________________________________________________________
block7c_expand_bn (BatchNormali (None, None, None, 3 12288 block7c_expand_conv[0][0]
__________________________________________________________________________________________________
block7c_expand_activation (Acti (None, None, None, 3 0 block7c_expand_bn[0][0]
__________________________________________________________________________________________________
block7c_dwconv (DepthwiseConv2D (None, None, None, 3 27648 block7c_expand_activation[0][0]
__________________________________________________________________________________________________
block7c_bn (BatchNormalization) (None, None, None, 3 12288 block7c_dwconv[0][0]
__________________________________________________________________________________________________
block7c_activation (Activation) (None, None, None, 3 0 block7c_bn[0][0]
__________________________________________________________________________________________________
block7c_se_squeeze (GlobalAvera (None, 3072) 0 block7c_activation[0][0]
__________________________________________________________________________________________________
block7c_se_reshape (Reshape) (None, 1, 1, 3072) 0 block7c_se_squeeze[0][0]
__________________________________________________________________________________________________
block7c_se_reduce (Conv2D) (None, 1, 1, 128) 393344 block7c_se_reshape[0][0]
__________________________________________________________________________________________________
block7c_se_expand (Conv2D) (None, 1, 1, 3072) 396288 block7c_se_reduce[0][0]
__________________________________________________________________________________________________
block7c_se_excite (Multiply) (None, None, None, 3 0 block7c_activation[0][0]
block7c_se_expand[0][0]
__________________________________________________________________________________________________
block7c_project_conv (Conv2D) (None, None, None, 5 1572864 block7c_se_excite[0][0]
__________________________________________________________________________________________________
block7c_project_bn (BatchNormal (None, None, None, 5 2048 block7c_project_conv[0][0]
__________________________________________________________________________________________________
block7c_drop (FixedDropout) (None, None, None, 5 0 block7c_project_bn[0][0]
__________________________________________________________________________________________________
block7c_add (Add) (None, None, None, 5 0 block7c_drop[0][0]
block7b_add[0][0]
__________________________________________________________________________________________________
top_conv (Conv2D) (None, None, None, 2 1048576 block7c_add[0][0]
__________________________________________________________________________________________________
top_bn (BatchNormalization) (None, None, None, 2 8192 top_conv[0][0]
__________________________________________________________________________________________________
top_activation (Activation) (None, None, None, 2 0 top_bn[0][0]
__________________________________________________________________________________________________
avg_pool (GlobalAveragePooling2 (None, 2048) 0 top_activation[0][0]
__________________________________________________________________________________________________
dropout (Dropout) (None, 2048) 0 avg_pool[0][0]
__________________________________________________________________________________________________
output (Dense) (None, 5) 10245 dropout[0][0]
==================================================================================================
Total params: 28,523,765
Trainable params: 28,351,029
Non-trainable params: 172,736
__________________________________________________________________________________________________
###Markdown
Test set predictions
###Code
files_path = f'{database_base_path}test_images/'
test_size = len(os.listdir(files_path))
test_preds = np.zeros((test_size, N_CLASSES))
for model_path in model_path_list:
print(model_path)
K.clear_session()
model.load_weights(model_path)
if TTA_STEPS > 0:
test_ds = get_dataset(files_path, tta=True).repeat()
ct_steps = TTA_STEPS * ((test_size/BATCH_SIZE) + 1)
preds = model.predict(test_ds, steps=ct_steps, verbose=1)[:(test_size * TTA_STEPS)]
preds = np.mean(preds.reshape(test_size, TTA_STEPS, N_CLASSES, order='F'), axis=1)
test_preds += preds / len(model_path_list)
else:
test_ds = get_dataset(files_path, tta=False)
x_test = test_ds.map(lambda image, image_name: image)
test_preds += model.predict(x_test) / len(model_path_list)
test_preds = np.argmax(test_preds, axis=-1)
test_names_ds = get_dataset(files_path)
image_names = [img_name.numpy().decode('utf-8') for img, img_name in iter(test_names_ds.unbatch())]
submission = pd.DataFrame({'image_id': image_names, 'label': test_preds})
submission.to_csv('submission.csv', index=False)
display(submission.head())
###Output
_____no_output_____ |
docs/notebooks/tut05_hsvd.ipynb | ###Markdown
5. Water suppression with HSVD In this tutorial we will take a look at water suppression. Water is present in the body at concentrations thousands of times higher than any of the metabolites we are interested in, so any spectrum where the water signal is not suppressed is completely dominated by the water peak centred at 4.7ppm.The standard way to suppress the water is to use the CHESS (chemical shift selective) technique. This preparation method uses a frequency selective excitation pulse to excite only the spins in the region of the water peak, followed by a "crusher" gradient pulse which dephases the excited spins. Once they have lost their phase coherence, these spins will no longer contribute any signal during the acquisition. In practice, the basic CHESS technique has been superseded by first WET and now VAPOR, which use a sequence of CHESS style pulses with varying flip angles and delays to achieve greater tolerance to B1 variation, and generally improved performance.However, in many cases, this prospective water suppression is insufficient to completely remove the water signal. Regions with poor shim, such as tumour, may have a water peak which partially extends outside the suppression region, and patient movement can have the same effect. Furthermore, many users choose to reduce the effect of water suppression by allowing a small amount of T1 recovery between the CHESS and the acquisition sequence. This approach, often referred to as "weak" water suppression, gives a large residual water peak which is useful during processing, for calculating channel weights and correcting frequency shifts. This peak must then be removed in a further processing step.The methods available for removing the residual water peak generally involve some form of bandpass filter which removes the signal from a particular region of the spectrum. For this tutorial we are going to focus on the most widely used technique, HSVD (Hankel Singular Value Decomposition).As usual, we start by importing our dependencies:
###Code
import suspect
import numpy as np
import matplotlib.pyplot as plt
%matplotlib inline
###Output
_____no_output_____
###Markdown
For this tutorial, we will be using the SVS_30.rda data included in the Suspect test data collection, so that we don't have to worry about channel combination or frequency correction here. However, we will repeat the apodisation step described in [Tutorial 1](tut01_intro.html).
###Code
data = suspect.io.load_rda("/home/jovyan/suspect/tests/test_data/siemens/SVS_30.rda")
import scipy.signal
window = scipy.signal.tukey(data.np * 2)[data.np:]
data = window * data
###Output
_____no_output_____
###Markdown
If we plot the raw spectrum we immediately see that the water peak completely dominates all the other peaks in the spectrum:
###Code
plt.plot(data.spectrum().real)
###Output
_____no_output_____
###Markdown
HSVD works by approximating the FID with a set of exponentially decaying components:
###Code
components = suspect.processing.water_suppression.hsvd(data, 20)
###Output
_____no_output_____
###Markdown
The second argument to the function is the number of components to generate. This will depend on both the number of peaks in the spectrum and how Lorentzian they are. Too few components will not be able to correctly describe the signal but too many can lead to over-fitting. Around 20 is typically a good number for most cases, but do experiment with your own data to understand better exactly what is going on.The `hsvd()` function returns a `list` of `dict`s, with each `dict` containing information about one exponential component:
###Code
print(components[0])
###Output
{'phase': -2.3526332899894427, 'amplitude': 137.25589213068457, 'fwhm': 0.93358884978978407, 'frequency': 598.57586504857886}
###Markdown
This components `list` can be turned back into an FID using the `construct_fid()` function, which takes a list of components to be used and a reference time axis. In this example we also set the resulting FID to `inherit()` all the MRS properties from the original `data` object.
###Code
hsvd_fid = suspect.processing.water_suppression.construct_fid(components, data.time_axis())
hsvd_fid = data.inherit(hsvd_fid)
# plot two axes, one of the whole spectrum and one focussing on the metabolite region
f, (ax1, ax2) = plt.subplots(2)
ax2.set_xlim([550, 850])
ax2.set_ylim([0, 2e5])
for ax in (ax1, ax2):
ax.plot(data.spectrum().real)
ax.plot(hsvd_fid.spectrum().real)
###Output
_____no_output_____
###Markdown
Overall we see that the `hsvd_fid` is a very good approximation to the original `data` signal, although some of the smaller peaks such as the Glx region are not fitted. To get a better idea of what is going on, we can reconstruct each component individually and plot the whole set together.
###Code
# plot two axes, one of the whole dataset and one of the metabolite region
f, (ax1, ax2) = plt.subplots(2)
ax2.set_xlim([550, 850])
ax2.set_ylim([-1e5, 5e5])
for component in components:
component_fid = suspect.processing.water_suppression.construct_fid([component], data.time_axis())
component_fid = data.inherit(component_fid)
ax1.plot(component_fid.spectrum().real)
ax2.plot(component_fid.spectrum().real)
###Output
_____no_output_____
###Markdown
What we find is that the major metabolite peaks each have one component associated with them, while the water peak has several. This is because it is not a perfect Lorentzian - to adequately describe the peak shape requires a series of progressively smaller correction terms to modify the main peak. Typically only the water peak gets multiple components as the others are too small, and the total number of components is limited.The next step is to separate out the components making up the water signal from the metabolite components, which we do using a frequency cut-off. We can do this rather neatly using a Python list comprehension:
###Code
water_components = [component for component in components if component["frequency"] < 70 or component["fwhm"] > 100]
###Output
_____no_output_____
###Markdown
In this case we have selected all the components with frequencies below 80Hz. The best value for this cut-off frequency will depend strongly on your data, and of course on the field strength of the magnet, but 80Hz is a reasonable starting point for most people at 3T. For our data we don't have any peaks downfield of water so we don't need a negative frequency cut-off.In addition we have selected a second set of components, this time with a FWHM greater than 100Hz. These very broad components are part of the baseline and it can be helpful to remove these at the same time.Once we have selected the components we want to remove, we can simply subtract the constructed FIDs from our original data to arrive at the water suppressed spectrum.
###Code
water_fid = suspect.processing.water_suppression.construct_fid(water_components, data.time_axis())
water_fid = data.inherit(water_fid)
dry_fid = data - water_fid
# plot two axes, one of the whole spectrum and one focussing on the metabolite region
f, (ax1, ax2) = plt.subplots(2)
ax2.set_xlim([550, 850])
ax2.set_ylim([-1e5, 2e5])
for ax in (ax1, ax2):
ax.plot(data.spectrum().real)
ax.plot(water_fid.spectrum().real)
ax.plot(dry_fid.spectrum().real)
###Output
_____no_output_____ |
src/static/misc/TF_lecture.ipynb | ###Markdown
CS285 Fall 2019 Tensorflow Tutorial This tutorial will provide a brief overview of the core concepts and functionality of Tensorflow. This tutorial will cover the following:0. What is Tensorflow1. How to input data2. How to perform computations3. How to create variables4. How to train a neural network for a simple regression problem5. Tips and tricks
###Code
# this just removes verbose warnings
import os
import warnings
os.environ['TF_CPP_MIN_LOG_LEVEL'] = '3'
warnings.filterwarnings('ignore')
import tensorflow as tf
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.cm as cm
import matplotlib.patches as mpatches
# for didactic purposes
def tf_reset():
try:
sess.close()
except:
pass
tf.reset_default_graph()
return tf.Session()
###Output
_____no_output_____
###Markdown
0. What is TensorflowTensorflow is a framework to define a series of computations. You define inputs, what operations should be performed, and then Tensorflow will compute the outputs for you.Below is a simple high-level example:
###Code
# create the session you'll work in
# you can think of this as a "blank piece of paper" that you'll be writing math on
sess = tf_reset()
# define your inputs
a = tf.constant(1.0)
b = tf.constant(2.0)
# do some operations
c = a + b
# get the result
c_run = sess.run(c)
print('c = {0}'.format(c_run))
###Output
_____no_output_____
###Markdown
1. How to input dataTensorflow has multiple ways for you to input data. One way is to have the inputs be constants:
###Code
sess = tf_reset()
# define your inputs
a = tf.constant(1.0)
b = tf.constant(2.0)
# do some operations
c = a + b
# get the result
c_run = sess.run(c)
print('c = {0}'.format(c_run))
###Output
_____no_output_____
###Markdown
However, having our inputs be constants is inflexible. We want to be able to change what data we input at runtime. We can do this using placeholders:
###Code
sess = tf_reset()
# define your inputs
a = tf.placeholder(dtype=tf.float32, shape=[1], name='a_placeholder')
b = tf.placeholder(dtype=tf.float32, shape=[1], name='b_placeholder')
# do some operations
c = a + b
# get the result
c0_run = sess.run(c, feed_dict={a: [1.0], b: [2.0]})
c1_run = sess.run(c, feed_dict={a: [2.0], b: [4.0]})
print('c0 = {0}'.format(c0_run))
print('c1 = {0}'.format(c1_run))
###Output
_____no_output_____
###Markdown
But what if we don't know the size of our input beforehand? One dimension of a tensor is allowed to be 'None', which means it can be variable sized:
###Code
sess = tf_reset()
# inputs
a = tf.placeholder(dtype=tf.float32, shape=[None], name='a_placeholder')
b = tf.placeholder(dtype=tf.float32, shape=[None], name='b_placeholder')
# do some operations
c = a + b
# get outputs
c0_run = sess.run(c, feed_dict={a: [1.0], b: [2.0]})
c1_run = sess.run(c, feed_dict={a: [1.0, 2.0], b: [2.0, 4.0]})
print(a)
print('a shape: {0}'.format(a.get_shape()))
print(b)
print('b shape: {0}'.format(b.get_shape()))
print('c0 = {0}'.format(c0_run))
print('c1 = {0}'.format(c1_run))
###Output
_____no_output_____
###Markdown
2. How to perform computationsNow that we can input data, we want to perform useful computations on the data. First, let's create some data to work with:
###Code
sess = tf_reset()
# inputs
a = tf.constant([[-1.], [-2.], [-3.]], dtype=tf.float32)
b = tf.constant([[1., 2., 3.]], dtype=tf.float32)
a_run, b_run = sess.run([a, b])
print('a:\n{0}'.format(a_run))
print('b:\n{0}'.format(b_run))
###Output
_____no_output_____
###Markdown
We can do simple operations, such as addition:
###Code
c = b + b
c_run = sess.run(c)
print('b:\n{0}'.format(b_run))
print('c:\n{0}'.format(c_run))
###Output
_____no_output_____
###Markdown
Be careful about the dimensions of the tensors, some operations may work even when you think they shouldn't...
###Code
c = a + b
c_run = sess.run(c)
print('a:\n{0}'.format(a_run))
print('b:\n{0}'.format(b_run))
print('c:\n{0}'.format(c_run))
###Output
_____no_output_____
###Markdown
Also, some operations may be different than what you expect:
###Code
c_elementwise = a * b
c_matmul = tf.matmul(b, a)
c_elementwise_run, c_matmul_run = sess.run([c_elementwise, c_matmul])
print('a:\n{0}'.format(a_run))
print('b:\n{0}'.format(b_run))
print('c_elementwise:\n{0}'.format(c_elementwise_run))
print('c_matmul: \n{0}'.format(c_matmul_run))
###Output
_____no_output_____
###Markdown
Operations can be chained together:
###Code
# operations can be chained together
c0 = b + b
c1 = c0 + 1
c0_run, c1_run = sess.run([c0, c1])
print('b:\n{0}'.format(b_run))
print('c0:\n{0}'.format(c0_run))
print('c1:\n{0}'.format(c1_run))
###Output
_____no_output_____
###Markdown
Finally, Tensorflow has many useful built-in operations:
###Code
c = tf.reduce_mean(b)
c_run = sess.run(c)
print('b:\n{0}'.format(b_run))
print('c:\n{0}'.format(c_run))
###Output
_____no_output_____
###Markdown
3. How to create variablesNow that we can input data and perform computations, we want some of these operations to involve variables that are free parameters, and can be trained using an optimizer (e.g., gradient descent). First, let's create some data to work with:
###Code
sess = tf_reset()
# inputs
b = tf.constant([[1., 2., 3.]], dtype=tf.float32)
sess = tf.Session()
b_run = sess.run(b)
print('b:\n{0}'.format(b_run))
###Output
_____no_output_____
###Markdown
We'll now create a variable
###Code
var_init_value = [[2.0, 4.0, 6.0]]
var = tf.get_variable(name='myvar',
shape=[1, 3],
dtype=tf.float32,
initializer=tf.constant_initializer(var_init_value))
print(var)
###Output
_____no_output_____
###Markdown
and check that it's been added to Tensorflow's variables list:
###Code
print(tf.global_variables())
###Output
_____no_output_____
###Markdown
We can do operations with the variable just like any other tensor:
###Code
# can do operations
c = b + var
print(b)
print(var)
print(c)
###Output
_____no_output_____
###Markdown
Before we can run any of these operations, we must first initalize the variables
###Code
init_op = tf.global_variables_initializer()
sess.run(init_op)
###Output
_____no_output_____
###Markdown
and then we can run the operations just as we normally would.
###Code
c_run = sess.run(c)
print('b:\n{0}'.format(b_run))
print('var:\n{0}'.format(var_init_value))
print('c:\n{0}'.format(c_run))
###Output
_____no_output_____
###Markdown
So far we haven't said yet how to optimize these variables. We'll cover that next in the context of an example. 4. How to train a neural network for a simple regression problemWe've discussed how to input data, perform operations, and create variables. We'll now show how to combine all of these---with some minor additions---to train a neural network on a simple regression problem. First, we'll create data for a 1-dimensional regression problem:
###Code
# generate the data
inputs = np.linspace(-2*np.pi, 2*np.pi, 10000)[:, None]
outputs = np.sin(inputs) + 0.05 * np.random.normal(size=[len(inputs),1])
plt.scatter(inputs[:, 0], outputs[:, 0], s=0.1, color='k', marker='o')
###Output
_____no_output_____
###Markdown
The below code creates the inputs, variables, neural network operations, mean-squared-error loss, gradient descent optimizer, and runs the optimizer using minibatches of the data.
###Code
sess = tf_reset()
def create_model():
# create inputs
input_ph = tf.placeholder(dtype=tf.float32, shape=[None, 1])
output_ph = tf.placeholder(dtype=tf.float32, shape=[None, 1])
# create variables
W0 = tf.get_variable(name='W0', shape=[1, 20], initializer=tf.contrib.layers.xavier_initializer())
W1 = tf.get_variable(name='W1', shape=[20, 20], initializer=tf.contrib.layers.xavier_initializer())
W2 = tf.get_variable(name='W2', shape=[20, 1], initializer=tf.contrib.layers.xavier_initializer())
b0 = tf.get_variable(name='b0', shape=[20], initializer=tf.constant_initializer(0.))
b1 = tf.get_variable(name='b1', shape=[20], initializer=tf.constant_initializer(0.))
b2 = tf.get_variable(name='b2', shape=[1], initializer=tf.constant_initializer(0.))
weights = [W0, W1, W2]
biases = [b0, b1, b2]
activations = [tf.nn.relu, tf.nn.relu, None]
# create computation graph
layer = input_ph
for W, b, activation in zip(weights, biases, activations):
layer = tf.matmul(layer, W) + b
if activation is not None:
layer = activation(layer)
output_pred = layer
return input_ph, output_ph, output_pred
input_ph, output_ph, output_pred = create_model()
# create loss
mse = tf.reduce_mean(0.5 * tf.square(output_pred - output_ph))
# create optimizer
opt = tf.train.AdamOptimizer().minimize(mse)
# initialize variables
sess.run(tf.global_variables_initializer())
# create saver to save model variables
saver = tf.train.Saver()
# run training
batch_size = 32
for training_step in range(10000):
# get a random subset of the training data
indices = np.random.randint(low=0, high=len(inputs), size=batch_size)
input_batch = inputs[indices]
output_batch = outputs[indices]
# run the optimizer and get the mse
_, mse_run = sess.run([opt, mse], feed_dict={input_ph: input_batch, output_ph: output_batch})
# print the mse every so often
if training_step % 1000 == 0:
print('{0:04d} mse: {1:.3f}'.format(training_step, mse_run))
saver.save(sess, '/tmp/model.ckpt')
###Output
_____no_output_____
###Markdown
Now that the neural network is trained, we can use it to make predictions:
###Code
sess = tf_reset()
# create the model
input_ph, output_ph, output_pred = create_model()
# restore the saved model
saver = tf.train.Saver()
saver.restore(sess, "/tmp/model.ckpt")
output_pred_run = sess.run(output_pred, feed_dict={input_ph: inputs})
plt.scatter(inputs[:, 0], outputs[:, 0], c='k', marker='o', s=0.1)
plt.scatter(inputs[:, 0], output_pred_run[:, 0], c='r', marker='o', s=0.1)
###Output
_____no_output_____
###Markdown
Not so hard after all! There is much more functionality to Tensorflow besides what we've covered, but you now know the basics. 5. Tips and tricks (a) Check your dimensions
###Code
# example of "surprising" resulting dimensions due to broadcasting
a = tf.constant(np.random.random((4, 1)))
b = tf.constant(np.random.random((1, 4)))
c = a * b
assert c.get_shape() == (4, 4)
###Output
_____no_output_____
###Markdown
(b) Check what variables have been created
###Code
sess = tf_reset()
a = tf.get_variable('I_am_a_variable', shape=[4, 6])
b = tf.get_variable('I_am_a_variable_too', shape=[2, 7])
for var in tf.global_variables():
print(var.name)
###Output
_____no_output_____
###Markdown
(c) Look at the [tensorflow API](https://www.tensorflow.org/api_docs/python/), or open up a python terminal and investigate!
###Code
help(tf.reduce_mean)
###Output
_____no_output_____
###Markdown
(d) Tensorflow has some built-in layers to simplify your code.
###Code
help(tf.contrib.layers.fully_connected)
###Output
_____no_output_____
###Markdown
(e) Use [variable scope](https://www.tensorflow.org/guide/variablessharing_variables) to keep your variables organized.
###Code
sess = tf_reset()
# create variables
with tf.variable_scope('layer_0'):
W0 = tf.get_variable(name='W0', shape=[1, 20], initializer=tf.contrib.layers.xavier_initializer())
b0 = tf.get_variable(name='b0', shape=[20], initializer=tf.constant_initializer(0.))
with tf.variable_scope('layer_1'):
W1 = tf.get_variable(name='W1', shape=[20, 20], initializer=tf.contrib.layers.xavier_initializer())
b1 = tf.get_variable(name='b1', shape=[20], initializer=tf.constant_initializer(0.))
with tf.variable_scope('layer_2'):
W2 = tf.get_variable(name='W2', shape=[20, 1], initializer=tf.contrib.layers.xavier_initializer())
b2 = tf.get_variable(name='b2', shape=[1], initializer=tf.constant_initializer(0.))
# print the variables
var_names = sorted([v.name for v in tf.global_variables()])
print('\n'.join(var_names))
###Output
_____no_output_____
###Markdown
(f) You can specify which GPU you want to use and how much memory you want to use
###Code
gpu_device = 0
gpu_frac = 0.5
# make only one of the GPUs visible
import os
os.environ["CUDA_VISIBLE_DEVICES"] = str(gpu_device)
# only use part of the GPU memory
gpu_options = tf.GPUOptions(per_process_gpu_memory_fraction=gpu_frac)
config = tf.ConfigProto(gpu_options=gpu_options)
# create the session
tf_sess = tf.Session(graph=tf.Graph(), config=config)
###Output
_____no_output_____ |
RandomForrest.ipynb | ###Markdown
Training Model
###Code
# Pandas is used for data manipulation
import pandas as pd
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
%matplotlib inline
# Read in data as a dataframe
df = pd.read_csv('data1_failure_ef.csv')
da=df
da=da[['dummy_activity_3','trans_id','trn_ct1',
'trn_ct2',
'trn_ct3',
'trn_ct4','trans_count', 'dep_amt1',
'dep_amt2',
'dep_amt3',
'dep_amt4','with_amt1',
'with_amt2',
'with_amt3','hour_day1',
'hour_day2',
'hour_day3',
'hour_day4',
'hour_day5',
'hour_day6',
'hour_day7',
'hour_day8',
'hour_day9',
'hour_day10',
'hour_day11',
'hour_day12',
'hour_day13',
'hour_day14',
'hour_day15',
'hour_day16',
'hour_day17',
'hour_day18',
'hour_day19',
'hour_day20',
'hour_day21',
'hour_day22',
'hour_day23',
'hour_day24',
'bco_retailoutletdetails1',
'bco_retailoutletdetails2',
'bco_retailoutletdetails3',
'bco_retailoutletdetails4',
'bco_retailoutletdetails5',
'bco_retailoutletdetails6',
'bco_retailoutletdetails7',
'bco_retailoutletdetails8',
'bco_retailoutletdetails9',
'bco_retailoutletdetails10',
'bco_retailoutletdetails11',
'bco_retailoutletdetails12',
'bco_retailoutletdetails13',
'bco_retailoutletdetails14',
'bco_retailoutletdetails15',
'bco_retailoutletdetails16',
'bco_retailoutletdetails17',
'bco_retailoutletdetails18',
'bco_retailoutletdetails19',
'bco_retailoutletdetails20',
'bco_retailoutletdetails21',
'bco_retailoutletdetails22',
'bco_retailoutletdetails23',
'bco_retailoutletdetails24',
'bco_retailoutletdetails25',
'bco_retailoutletdetails26',
'bco_retailoutletdetails27',
'bco_retailoutletdetails28',
'bco_retailoutletdetails29',
'bco_retailoutletdetails30',
'bco_retailoutletdetails31',
'bco_retailoutletdetails32',
'bco_retailoutletdetails33',
'bco_retailoutletdetails34',
'bco_retailoutletdetails35',
'bco_retailoutletdetails36',
'bco_retailoutletdetails37',
'bco_retailoutletdetails38',
'bco_retailoutletdetails39',
'bco_retailoutletdetails40',
'bco_retailoutletdetails41',
'bco_retailoutletdetails42',
'bco_retailoutletdetails43',
'bco_retailoutletdetails44',
'bco_retailoutletdetails45',
'bco_retailoutletdetails46',
'bco_retailoutletdetails47',
'bco_retailoutletdetails48',
'bco_retailoutletdetails49',
'bco_retailoutletdetails50',
'bco_retailoutletdetails51',
'bco_retailoutletdetails52',
'bco_retailoutletdetails53',
'bco_retailoutletdetails54',
'bco_retailoutletdetails55',
'bco_retailoutletdetails56',
'bco_retailoutletdetails57',
'bco_retailoutletdetails58',
'bco_retailoutletdetails59',
'bco_retailoutletdetails60', 'age_customer',
'years_experience',
'yrs_exp1',
'yrs_exp2',
'yrs_exp3',
'yrs_exp4',
'ag_cust1',
'ag_cust2',
'ag_cust3',
'ag_cust4',
'month1',
'month2',
'month3',
'month4',
'month5',
'month6',
'month7',
'month8',
'month9',
'month10',
'month11',
'month12',
'dow1',
'dow2',
'dow3',
'dow4',
'dow5',
'dow6',
'dow7',
'dummy_failure',
'dummy_balanceinquiry',
'dummy_ministatement',
'dummy_working_hours',
'dummy_biometric',
'dummy_technical_fail',
'dummy_non_technical_fail',
'deposit_amount',
'withdrawal_amount',
'town_village1',
'town_village2',
'town_village3',
'town_village4',
'town_village5',
'town_village6',
'town_village7',
'town_village8',
'town_village9',
'town_village10',
'town_village11',
'town_village12',
'town_village13',
'town_village14',
'town_village15',
'town_village16',
'town_village17',
'town_village18',
'town_village19',
'town_village20',
'town_village21',
'town_village22',
'town_village23',
'town_village24',
'town_village25',
'town_village26',
'town_village27',
'town_village28',
'town_village29',
'town_village30',
'town_village31',
'town_village32',
'town_village33',
'town_village34',
'town_village35',
'town_village36',
'town_village37',
'town_village38',
'town_village39',
'town_village40',
'town_village41',
'town_village42',
'town_village43',
'town_village44',
'town_village45',
'town_village46',
'town_village47',
'town_village48',
'town_village49',
'town_village50',
'town_village51',
'town_village52',
'town_village53',
'town_village54',
'town_village55',
'town_village56',
'town_village57',
'town_village58',
'town_village59',
'town_village60',
'town_village61',
'town_village62',
'town_village63',
'town_village64',
'town_village65',
'town_village66',
'town_village67',
'town_village68',
'town_village69',
'town_village70',
'town_village71',
'town_village72',
'town_village73',
'town_village74',
'town_village75',
'town_village76',
'town_village77',
'town_village78',
'town_village79',
'town_village80',
'town_village81',
'town_village82',
'town_village83',
'town_village84',
'town_village85',
'town_village86',
'town_village87',
'town_village88',
'town_village89',
'town_village90',
'town_village91',
'town_village92',
'town_village93',
'town_village94',
'town_village95',
'town_village96',
'town_village97',
'town_village98',
'town_village99',
'town_village100',
'town_village101',
'town_village102',
'town_village103',
'town_village104',
'town_village105',
'town_village106',
'town_village107',
'town_village108',
'town_village109',
'town_village110',
'town_village111',
'town_village112',
'town_village113',
'town_village114',
'town_village115',
'town_village116',
'town_village117',
'town_village118',
'town_village119',
'town_village120',
'town_village121',
'town_village122',
'town_village123',
'town_village124',
'town_village125',
'town_village126',
'town_village127',
'town_village128',
'town_village129',
'town_village130',
'town_village131',
'town_village132',
'town_village133',
'town_village134',
'town_village135',
'town_village136',
'town_village137',
'town_village138',
'town_village139',
'town_village140',
'town_village141',
'town_village142',
'town_village143',
'town_village144',
'town_village145',
'town_village146',
'town_village147',
'town_village148',
'town_village149',
'town_village150',
'town_village151',
'town_village152',
'town_village153',
'town_village154',
'town_village155',
'town_village156',
'town_village157',
'town_village158',
'town_village159',
'town_village160',
'town_village161',
'town_village162',
'town_village163',
'town_village164',
'town_village165',
'town_village166',
'town_village167',
'town_village168',
'town_village169',
'town_village170',
'town_village171',
'town_village172',
'town_village173',
'town_village174',
'town_village175',
'town_village176',
'town_village177',
'town_village178',
'town_village179',
'town_village180',
'town_village181',
'town_village182',
'town_village183',
'town_village184',
'town_village185',
'town_village186',
'town_village187',
'town_village188',
'town_village189',
'town_village190',
'town_village191',
'town_village192',
'town_village193',
'town_village194',
'town_village195',
'town_village196',
'town_village197',
'town_village198',
'town_village199',
'town_village200',
'town_village201',
'town_village202',
'town_village203',
'town_village204',
'town_village205',
'town_village206',
'town_village207',
'town_village208',
'town_village209',
'town_village210',
'town_village211',
'town_village212',
'town_village213',
'town_village214',
'town_village215',
'town_village216',
'town_village217',
'town_village218',
'town_village219',
'town_village220',
'town_village221',
'town_village222',
'town_village223',
'town_village224',
'town_village225',
'town_village226',
'town_village227',
'town_village228',
'town_village229',
'town_village230',
'town_village231',
'town_village232',
'town_village233',
'town_village234',
'town_village235',
'town_village236',
'town_village237',
'town_village238',
'town_village239',
'town_village240',
'town_village241',
'town_village242',
'town_village243',
'town_village244',
'town_village245',
'town_village246',
'town_village247',
'town_village248',
'town_village249',
'town_village250',
'town_village251',
'town_village252',
'town_village253',
'town_village254',
'town_village255',
'town_village256',
'town_village257',
'town_village258',
'town_village259',
'town_village260',
'town_village261',
'town_village262',
'town_village263',
'town_village264',
'town_village265',
'town_village266',
'town_village267',
'town_village268',
'town_village269',
'town_village270',
'town_village271',
'town_village272',
'town_village273',
'town_village274',
'town_village275',
'town_village276',
'town_village277',
'town_village278',
'town_village279',
'town_village280',
'town_village281',
'town_village282',
'town_village283',
'town_village284',
'town_village285',
'town_village286',
'town_village287',
'town_village288',
'town_village289',
'town_village290',
'town_village291',
'town_village292',
'town_village293',
'town_village294',
'town_village295',
'town_village296',
'town_village297',
'town_village298',
'town_village299',
'town_village300',
'town_village301',
'town_village302',
'town_village303',
'town_village304',
'town_village305',
'town_village306',
'town_village307',
'town_village308',
'town_village309',
'town_village310',
'town_village311',
'town_village312',
'town_village313',
'town_village314',
'town_village315',
'town_village316',
'town_village317',
'town_village318',
'town_village319',
'town_village320',
'town_village321',
'town_village322',
'town_village323',
'town_village324',
'town_village325',
'town_village326',
'town_village327',
'town_village328',
'town_village329',
'town_village330',
'town_village331',
'town_village332',
'town_village333',
'town_village334',
'town_village335',
'town_village336',
'town_village337',
'town_village338',
'town_village339',
'town_village340',
'town_village341',
'town_village342',
'town_village343',
'town_village344',
'town_village345',
'town_village346',
'town_village347',
'town_village348',
'town_village349',
'town_village350',
'town_village351',
'town_village352',
'town_village353',
'town_village354',
'town_village355',
'town_village356',
'town_village357',
'town_village358',
'town_village359',
'town_village360',
'town_village361',
'town_village362',
'town_village363',
'town_village364',
'town_village365',
'town_village366',
'town_village367',
'town_village368',
'town_village369',
'town_village370',
'town_village371',
'town_village372',
'town_village373',
'town_village374',
'town_village375',
'town_village376',
'town_village377',
'town_village378',
'town_village379',
'town_village380',
'town_village381',
'town_village382',
'town_village383',
'town_village384',
'town_village385',
'town_village386',
'town_village387',
'town_village388',
'town_village389',
'town_village390',
'town_village391',
'town_village392',
'town_village393',
'town_village394',
'town_village395',
'town_village396',
'town_village397',
'town_village398',
'town_village399',
'town_village400',
'town_village401',
'town_village402',
'town_village403',
'town_village404',
'town_village405',
'town_village406',
'town_village407',
'town_village408',
'town_village409',
'town_village410',
'town_village411',
'town_village412',
'town_village413',
'town_village414',
'town_village415',
'town_village416',
'town_village417',
'town_village418',
'town_village419',
'town_village420',
'town_village421',
'town_village422',
'town_village423']]
X = da.iloc[:, 2:]
y = da.iloc[:, 0]
###Output
_____no_output_____
###Markdown
Train and Test Split
###Code
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size = 0.03, random_state = 0)
###Output
_____no_output_____
###Markdown
Data Standardization
###Code
from sklearn.preprocessing import StandardScaler
sc_X = StandardScaler()
X_train = sc_X.fit_transform(X_train)
X_test = sc_X.transform(X_test)
###Output
_____no_output_____
###Markdown
Fit to Random Forest
###Code
sample_leaf_options = [10]
for leaf_size in sample_leaf_options :
from sklearn.ensemble import RandomForestClassifier
classifier = RandomForestClassifier(n_estimators=leaf_size, criterion='entropy', random_state=0,n_jobs = -1,min_samples_leaf = 1)
classifier.fit(X_train, y_train)
threshold = 0.4
predicted_proba = classifier.predict_proba(X_test)
predicted = (predicted_proba [:,1] >= threshold).astype('int')
from sklearn.metrics import accuracy_score
accuracy_xgb = accuracy_score(y_test,predicted)
print (accuracy_xgb)
from sklearn.metrics import confusion_matrix
cm = confusion_matrix(y_test, predicted)
cm
###Output
_____no_output_____
###Markdown
TEST
###Code
df1 = pd.read_csv('sample.csv')
da1=df1
df1
da1=da1[['trans_id','bc_id','cust_id','trn_ct1',
'trn_ct2',
'trn_ct3',
'trn_ct4','trans_count', 'dep_amt1',
'dep_amt2',
'dep_amt3',
'dep_amt4','with_amt1',
'with_amt2',
'with_amt3','hour_day1',
'hour_day2',
'hour_day3',
'hour_day4',
'hour_day5',
'hour_day6',
'hour_day7',
'hour_day8',
'hour_day9',
'hour_day10',
'hour_day11',
'hour_day12',
'hour_day13',
'hour_day14',
'hour_day15',
'hour_day16',
'hour_day17',
'hour_day18',
'hour_day19',
'hour_day20',
'hour_day21',
'hour_day22',
'hour_day23',
'hour_day24',
'bco_retailoutletdetails1',
'bco_retailoutletdetails2',
'bco_retailoutletdetails3',
'bco_retailoutletdetails4',
'bco_retailoutletdetails5',
'bco_retailoutletdetails6',
'bco_retailoutletdetails7',
'bco_retailoutletdetails8',
'bco_retailoutletdetails9',
'bco_retailoutletdetails10',
'bco_retailoutletdetails11',
'bco_retailoutletdetails12',
'bco_retailoutletdetails13',
'bco_retailoutletdetails14',
'bco_retailoutletdetails15',
'bco_retailoutletdetails16',
'bco_retailoutletdetails17',
'bco_retailoutletdetails18',
'bco_retailoutletdetails19',
'bco_retailoutletdetails20',
'bco_retailoutletdetails21',
'bco_retailoutletdetails22',
'bco_retailoutletdetails23',
'bco_retailoutletdetails24',
'bco_retailoutletdetails25',
'bco_retailoutletdetails26',
'bco_retailoutletdetails27',
'bco_retailoutletdetails28',
'bco_retailoutletdetails29',
'bco_retailoutletdetails30',
'bco_retailoutletdetails31',
'bco_retailoutletdetails32',
'bco_retailoutletdetails33',
'bco_retailoutletdetails34',
'bco_retailoutletdetails35',
'bco_retailoutletdetails36',
'bco_retailoutletdetails37',
'bco_retailoutletdetails38',
'bco_retailoutletdetails39',
'bco_retailoutletdetails40',
'bco_retailoutletdetails41',
'bco_retailoutletdetails42',
'bco_retailoutletdetails43',
'bco_retailoutletdetails44',
'bco_retailoutletdetails45',
'bco_retailoutletdetails46',
'bco_retailoutletdetails47',
'bco_retailoutletdetails48',
'bco_retailoutletdetails49',
'bco_retailoutletdetails50',
'bco_retailoutletdetails51',
'bco_retailoutletdetails52',
'bco_retailoutletdetails53',
'bco_retailoutletdetails54',
'bco_retailoutletdetails55',
'bco_retailoutletdetails56',
'bco_retailoutletdetails57',
'bco_retailoutletdetails58',
'bco_retailoutletdetails59',
'bco_retailoutletdetails60', 'age_customer',
'years_experience',
'yrs_exp1',
'yrs_exp2',
'yrs_exp3',
'yrs_exp4',
'ag_cust1',
'ag_cust2',
'ag_cust3',
'ag_cust4',
'month1',
'month2',
'month3',
'month4',
'month5',
'month6',
'month7',
'month8',
'month9',
'month10',
'month11',
'month12',
'dow1',
'dow2',
'dow3',
'dow4',
'dow5',
'dow6',
'dow7',
'dummy_failure',
'dummy_balanceinquiry',
'dummy_ministatement',
'dummy_working_hours',
'dummy_biometric',
'dummy_technical_fail',
'dummy_non_technical_fail',
'deposit_amount',
'withdrawal_amount',
'town_village1',
'town_village2',
'town_village3',
'town_village4',
'town_village5',
'town_village6',
'town_village7',
'town_village8',
'town_village9',
'town_village10',
'town_village11',
'town_village12',
'town_village13',
'town_village14',
'town_village15',
'town_village16',
'town_village17',
'town_village18',
'town_village19',
'town_village20',
'town_village21',
'town_village22',
'town_village23',
'town_village24',
'town_village25',
'town_village26',
'town_village27',
'town_village28',
'town_village29',
'town_village30',
'town_village31',
'town_village32',
'town_village33',
'town_village34',
'town_village35',
'town_village36',
'town_village37',
'town_village38',
'town_village39',
'town_village40',
'town_village41',
'town_village42',
'town_village43',
'town_village44',
'town_village45',
'town_village46',
'town_village47',
'town_village48',
'town_village49',
'town_village50',
'town_village51',
'town_village52',
'town_village53',
'town_village54',
'town_village55',
'town_village56',
'town_village57',
'town_village58',
'town_village59',
'town_village60',
'town_village61',
'town_village62',
'town_village63',
'town_village64',
'town_village65',
'town_village66',
'town_village67',
'town_village68',
'town_village69',
'town_village70',
'town_village71',
'town_village72',
'town_village73',
'town_village74',
'town_village75',
'town_village76',
'town_village77',
'town_village78',
'town_village79',
'town_village80',
'town_village81',
'town_village82',
'town_village83',
'town_village84',
'town_village85',
'town_village86',
'town_village87',
'town_village88',
'town_village89',
'town_village90',
'town_village91',
'town_village92',
'town_village93',
'town_village94',
'town_village95',
'town_village96',
'town_village97',
'town_village98',
'town_village99',
'town_village100',
'town_village101',
'town_village102',
'town_village103',
'town_village104',
'town_village105',
'town_village106',
'town_village107',
'town_village108',
'town_village109',
'town_village110',
'town_village111',
'town_village112',
'town_village113',
'town_village114',
'town_village115',
'town_village116',
'town_village117',
'town_village118',
'town_village119',
'town_village120',
'town_village121',
'town_village122',
'town_village123',
'town_village124',
'town_village125',
'town_village126',
'town_village127',
'town_village128',
'town_village129',
'town_village130',
'town_village131',
'town_village132',
'town_village133',
'town_village134',
'town_village135',
'town_village136',
'town_village137',
'town_village138',
'town_village139',
'town_village140',
'town_village141',
'town_village142',
'town_village143',
'town_village144',
'town_village145',
'town_village146',
'town_village147',
'town_village148',
'town_village149',
'town_village150',
'town_village151',
'town_village152',
'town_village153',
'town_village154',
'town_village155',
'town_village156',
'town_village157',
'town_village158',
'town_village159',
'town_village160',
'town_village161',
'town_village162',
'town_village163',
'town_village164',
'town_village165',
'town_village166',
'town_village167',
'town_village168',
'town_village169',
'town_village170',
'town_village171',
'town_village172',
'town_village173',
'town_village174',
'town_village175',
'town_village176',
'town_village177',
'town_village178',
'town_village179',
'town_village180',
'town_village181',
'town_village182',
'town_village183',
'town_village184',
'town_village185',
'town_village186',
'town_village187',
'town_village188',
'town_village189',
'town_village190',
'town_village191',
'town_village192',
'town_village193',
'town_village194',
'town_village195',
'town_village196',
'town_village197',
'town_village198',
'town_village199',
'town_village200',
'town_village201',
'town_village202',
'town_village203',
'town_village204',
'town_village205',
'town_village206',
'town_village207',
'town_village208',
'town_village209',
'town_village210',
'town_village211',
'town_village212',
'town_village213',
'town_village214',
'town_village215',
'town_village216',
'town_village217',
'town_village218',
'town_village219',
'town_village220',
'town_village221',
'town_village222',
'town_village223',
'town_village224',
'town_village225',
'town_village226',
'town_village227',
'town_village228',
'town_village229',
'town_village230',
'town_village231',
'town_village232',
'town_village233',
'town_village234',
'town_village235',
'town_village236',
'town_village237',
'town_village238',
'town_village239',
'town_village240',
'town_village241',
'town_village242',
'town_village243',
'town_village244',
'town_village245',
'town_village246',
'town_village247',
'town_village248',
'town_village249',
'town_village250',
'town_village251',
'town_village252',
'town_village253',
'town_village254',
'town_village255',
'town_village256',
'town_village257',
'town_village258',
'town_village259',
'town_village260',
'town_village261',
'town_village262',
'town_village263',
'town_village264',
'town_village265',
'town_village266',
'town_village267',
'town_village268',
'town_village269',
'town_village270',
'town_village271',
'town_village272',
'town_village273',
'town_village274',
'town_village275',
'town_village276',
'town_village277',
'town_village278',
'town_village279',
'town_village280',
'town_village281',
'town_village282',
'town_village283',
'town_village284',
'town_village285',
'town_village286',
'town_village287',
'town_village288',
'town_village289',
'town_village290',
'town_village291',
'town_village292',
'town_village293',
'town_village294',
'town_village295',
'town_village296',
'town_village297',
'town_village298',
'town_village299',
'town_village300',
'town_village301',
'town_village302',
'town_village303',
'town_village304',
'town_village305',
'town_village306',
'town_village307',
'town_village308',
'town_village309',
'town_village310',
'town_village311',
'town_village312',
'town_village313',
'town_village314',
'town_village315',
'town_village316',
'town_village317',
'town_village318',
'town_village319',
'town_village320',
'town_village321',
'town_village322',
'town_village323',
'town_village324',
'town_village325',
'town_village326',
'town_village327',
'town_village328',
'town_village329',
'town_village330',
'town_village331',
'town_village332',
'town_village333',
'town_village334',
'town_village335',
'town_village336',
'town_village337',
'town_village338',
'town_village339',
'town_village340',
'town_village341',
'town_village342',
'town_village343',
'town_village344',
'town_village345',
'town_village346',
'town_village347',
'town_village348',
'town_village349',
'town_village350',
'town_village351',
'town_village352',
'town_village353',
'town_village354',
'town_village355',
'town_village356',
'town_village357',
'town_village358',
'town_village359',
'town_village360',
'town_village361',
'town_village362',
'town_village363',
'town_village364',
'town_village365',
'town_village366',
'town_village367',
'town_village368',
'town_village369',
'town_village370',
'town_village371',
'town_village372',
'town_village373',
'town_village374',
'town_village375',
'town_village376',
'town_village377',
'town_village378',
'town_village379',
'town_village380',
'town_village381',
'town_village382',
'town_village383',
'town_village384',
'town_village385',
'town_village386',
'town_village387',
'town_village388',
'town_village389',
'town_village390',
'town_village391',
'town_village392',
'town_village393',
'town_village394',
'town_village395',
'town_village396',
'town_village397',
'town_village398',
'town_village399',
'town_village400',
'town_village401',
'town_village402',
'town_village403',
'town_village404',
'town_village405',
'town_village406',
'town_village407',
'town_village408',
'town_village409',
'town_village410',
'town_village411',
'town_village412',
'town_village413',
'town_village414',
'town_village415',
'town_village416',
'town_village417',
'town_village418',
'town_village419',
'town_village420',
'town_village421',
'town_village422',
'town_village423']]
X1 = da1.iloc[:, 3:]
y1 = da1.iloc[:, :3]
X1
X11=X1
input = sc_X.transform(X1)
threshold = 0.4
predicted_proba = classifier.predict_proba(input)
predicted = (predicted_proba [:,1] >= threshold).astype('int')
dataframe1=pd.DataFrame(predicted, columns=['output'])
df_out1=pd.concat([dataframe1,y1], axis=1)
df_out1.to_csv('input_final1.csv')
###Output
_____no_output_____ |
_build/html/_sources/contents/flow/lecture_05/L06-numericalcontent/Layered Systems.ipynb | ###Markdown
Layered Systems
###Code
import numpy as np
###Output
_____no_output_____
###Markdown
Case I - Flow Parallel to Layering
###Code
print("\033[1m\033[4mA quick example:\033[0m You can change the provided values.\n")
print("Calculate the effective hydraulic conductivity of the layer system consisting of 5 layers if the flow is parallel to the stratification.\n\n\033[1mProvided are:\033[0m")
#Thickness of i-th layer [m]
m1 = 3
m2 = 2.5
m3 = 1.75
#conductivity of i-th layer [m/s]
K1 = 3.5e-3
K2 = 2e-2
K3 = 5e-4
#intermediate calculation
m = m1+m2+m3
#solution
K = (m1*K1+m2*K2+m3*K3)/m
print("thickness of layer 1 = {}".format(m1), "m\nthickness of layer 2 = {}".format(m2),"m\nthickness of layer 3 = {}".format(m3), "m\nconductivity of layer 1 = {:02.1e}".format(K1),
"m/s\nconductivity of layer 2 = {:02.1e}".format(K2), "m/s\nconductivity of layer 3 {:02.1e}".format(K3), "m/s")
print("\n\033[1mSolution:\033[0m\nThe resulting hydraulic conductivity of the layer system is \033[1m{:02.1e} m/s\033[0m.".format(K))
###Output
[1m[4mA quick example:[0m You can change the provided values.
Calculate the effective hydraulic conductivity of the layer system consisting of 5 layers if the flow is parallel to the stratification.
[1mProvided are:[0m
thickness of layer 1 = 3 m
thickness of layer 2 = 2.5 m
thickness of layer 3 = 1.75 m
conductivity of layer 1 = 3.5e-03 m/s
conductivity of layer 2 = 2.0e-02 m/s
conductivity of layer 3 5.0e-04 m/s
[1mSolution:[0m
The resulting hydraulic conductivity of the layer system is [1m8.5e-03 m/s[0m.
###Markdown
Case II - Flow Perpendicular to Layering
###Code
print("\033[1m\033[4mA quick example:\033[0m You can change the provided values.\n")
print("Calculate the effective hydraulic conductivity of the layer system consisting of 5 layers if the flow is perpendicular to the layering.\n\n\033[1mProvided are:\033[0m")
#Thickness of i-th layer [m]
m1 = 3
m2 = 2.5
m3 = 1.75
#conductivity of i-th layer [m/s]
K1 = 3.5e-3
K2 = 2e-2
K3 = 5e-4
#intermediate calculation
m = m1+m2+m3
#solution
K = m/(m1/K1+m2/K2+m3/K3)
print("thickness of layer 1 = {}".format(m1), "m\nthickness of layer 2 = {}".format(m2),"m\nthickness of layer 3 = {}".format(m3), "m\nconductivity of layer 1 = {:02.1e}".format(K1),
"m/s\nconductivity of layer 2 = {:02.1e}".format(K2), "m/s\nconductivity of layer 3 {:02.1e}".format(K3), "m/s")
print("\n\033[1mSolution:\033[0m\nThe resulting hydraulic conductivity of the layer system is \033[1m{:02.1e} m/s\033[0m.".format(K))
###Output
[1m[4mA quick example:[0m You can change the provided values.
Calculate the effective hydraulic conductivity of the layer system consisting of 5 layers if the flow is perpendicular to the layering.
[1mProvided are:[0m
thickness of layer 1 = 3 m
thickness of layer 2 = 2.5 m
thickness of layer 3 = 1.75 m
conductivity of layer 1 = 3.5e-03 m/s
conductivity of layer 2 = 2.0e-02 m/s
conductivity of layer 3 5.0e-04 m/s
[1mSolution:[0m
The resulting hydraulic conductivity of the layer system is [1m1.6e-03 m/s[0m.
|
Jberkow Assignment 1 Software Engineering.ipynb | ###Markdown
###Code
pip install -i https://test.pypi.org/simple/ lambdata-jberkow713==1.3
from lambdata_jberkow713 import my_mod
import pandas as pd
import numpy as np
from lambdata_jberkow713.my_mod import shrink
from lambdata_jberkow713.my_mod import Berkofy
print(shrink(3))
Berkofy(8)
!pip install --upgrade autopep8
def shrink(n):
return int(n) * (1/n**3)
def Berkofy(n):
return int(n) * (n-1) + ((n-2)/(n-3))
###Output
_____no_output_____ |
Sample/Day_12_Sample.ipynb | ###Markdown
* 教學目標 * 知道 DataFrame 中迴圈的運作規則 * 了解 DataFrame 中 Map、 Apply、Applymap 差異 * 知道不建議在 DataFrame 進行迭代操作的原因
###Code
# 載入 NumPy, Pandas 套件
import numpy as np
import pandas as pd
# 檢查正確載入與版本
print(np)
print(np.__version__)
print(pd)
print(pd.__version__)
###Output
<module 'numpy' from 'D:\\anaconda3\\lib\\site-packages\\numpy\\__init__.py'>
1.19.2
<module 'pandas' from 'D:\\anaconda3\\lib\\site-packages\\pandas\\__init__.py'>
1.1.3
###Markdown
【基礎12】 DataFrame 當中的 For Loop
###Code
df = pd.DataFrame({
'name': ['Alice', 'Bob'],
'age': [20, 32]
})
for c in df:
print(c)
###Output
name
age
###Markdown
橫向的資料迭代如果我們想要對以「筆」為單位的資料迭代的話,最暴力的方法可以這樣做:
###Code
df = pd.DataFrame({
'name': ['Alice', 'Bob'],
'age': [20, 32]
})
for i in range(len(df)):
print(df.iloc[i])
###Output
name Alice
age 20
Name: 0, dtype: object
name Bob
age 32
Name: 1, dtype: object
###Markdown
iteritems()、iterrows()、itertuples()第二種方法可以直接用 DataFrame 內建的 iterative 方法:
###Code
for d in df.iteritems():
print(d)
for d in df.iterrows():
print(d)
for d in df.itertuples():
print(d)
###Output
('name', 0 Alice
1 Bob
Name: name, dtype: object)
('age', 0 20
1 32
Name: age, dtype: int64)
(0, name Alice
age 20
Name: 0, dtype: object)
(1, name Bob
age 32
Name: 1, dtype: object)
Pandas(Index=0, name='Alice', age=20)
Pandas(Index=1, name='Bob', age=32)
###Markdown
apply第三種方法是使用 Pandas 當中的 apply 方法,apply 是一種用於逐行或逐列的循環處理方法,常搭配 lambda 匿名函式一起使用:
###Code
df = pd.DataFrame({
'score': [98, 67, 85],
'age': [20, 32, 28]
})
print(df.apply(np.max))
print('='*20)
print(df.apply(np.min))
print('='*20)
print(df.apply(lambda x: x.max() - x.min()))
###Output
score 98
age 32
dtype: int64
====================
score 67
age 20
dtype: int64
====================
score 31
age 12
dtype: int64
###Markdown
map另外一種跟 apply 很像的方法叫做 map:
###Code
df = pd.DataFrame({
'score': [98, 67, 85],
'age': [20, 32, 28]
})
df['age'].map(lambda x: -x)
###Output
_____no_output_____
###Markdown
applymap在 Pandas 當中,有一種同時 apply 和 map 方法稱為 applymap:
###Code
df = pd.DataFrame({
'score': [98, 67, 85],
'age': [20, 32, 28]
})
df.applymap(lambda x: -x)
###Output
_____no_output_____
###Markdown
Map、 Apply、Applymap * map:對 series 所有元素作一樣的操作 * apply:對 series 或 dataframe 逐行或逐列做一樣的操作* applymap:對 dataframe 所有元素作一樣的操作 補充:lambda 匿名函式Map、 Apply、Applymap 很常搭配 lambda 匿名函式 一起使用,但其實裡面也可以放函式名稱,我們來比較看看:
###Code
df.apply(lambda x: x.max() - x.min())
def f(x):
return x.max() - x.min()
print(df.apply(f))
###Output
score 31
age 12
dtype: int64
|
examples/notebooks/example-usage.ipynb | ###Markdown
Example usage for `weightedcalcs`The example below uawa `weightedcalcs` to analyze a slice of the [American Community Survey's 2015 data](https://www.census.gov/programs-surveys/acs/technical-documentation/pums/documentation.html) for Wyoming.
###Code
import weightedcalcs as wc
import pandas as pd
###Output
_____no_output_____
###Markdown
Load the ACS data into a `pandas.DataFrame`
###Code
responses = pd.read_csv("../data/acs-2015-pums-wy-simple.csv")
responses.head()
###Output
_____no_output_____
###Markdown
In addition to the full list of responses, let's create a subset including only adult respondents, since we'll be focusing on income later.
###Code
adults = responses[responses["age"] >= 18]
adults.head()
###Output
_____no_output_____
###Markdown
Create an instance of `weightedcalcs.Calculator`The ACS' `PWGTP` variable is respondents the Census-assigned survey weight. All our weighted calculations will use this variable.
###Code
calc = wc.Calculator("PWGTP")
###Output
_____no_output_____
###Markdown
Basic weighted calculations Weighted mean income
###Code
calc.mean(adults, "income").round()
###Output
_____no_output_____
###Markdown
Weighted standard deviation of income
###Code
calc.std(adults, "income").round()
###Output
_____no_output_____
###Markdown
Weighted median income
###Code
calc.median(adults, "income")
###Output
_____no_output_____
###Markdown
Weighted 75th percentile of income
###Code
calc.quantile(adults, "income", 0.75)
###Output
_____no_output_____
###Markdown
Weighted distribution of marriage statuses ~43% of Wyoming residents are married:
###Code
calc.distribution(responses, "marriage_status").round(3).sort_values(ascending=False)
###Output
_____no_output_____
###Markdown
~56% of *adult* Wyoming residents are married:
###Code
calc.distribution(adults, "marriage_status").round(3).sort_values(ascending=False)
###Output
_____no_output_____
###Markdown
Grouped weighted calculationsBelow, we perform similar calculations as above, but now take advantage of the fact that `weightedcalcs` can handle `DataFrameGroupBy` objects. In the examples below, we group by the ACS's marriage status categories and gender.
###Code
grp_marriage_sex = adults.groupby(["marriage_status", "gender"])
###Output
_____no_output_____
###Markdown
For reference, here's how many responses fall into each category:
###Code
grp_marriage_sex.size().unstack()
###Output
_____no_output_____
###Markdown
Weighted mean income
###Code
calc.mean(grp_marriage_sex, "income").round().astype(int)
###Output
_____no_output_____
###Markdown
Weighted standard deviation of income
###Code
calc.std(grp_marriage_sex, "income").round()
###Output
_____no_output_____
###Markdown
Weighted median income
###Code
calc.median(grp_marriage_sex, "income")
###Output
_____no_output_____
###Markdown
Weighted 75th percentile of income
###Code
calc.quantile(grp_marriage_sex, "income", 0.75)
###Output
_____no_output_____ |
beta/load_dataset_second_time.ipynb | ###Markdown
Load DatasetTo clear all record and load all images to the /dataset.svg_w=960, svg_h=540
###Code
from app.models import Label,Image,Batch, Comment, STATUS_CHOICES
from django.contrib.auth.models import User
import os, fnmatch, uuid, shutil
from uuid import uuid4
def getbatchlist(filelist):
def chunks(li, n):
"""Yield successive n-sized chunks from l."""
for i in range(0, len(li), n):
yield li[i:i + n]
return list(chunks(filelist, 5))
print getbatchlist(range(10))
###Output
[[0, 1, 2, 3, 4], [5, 6, 7, 8, 9]]
###Markdown
FOR DEBUG ONLY !!!! Clear all batches and move images from /dataset to /rawprint "DELETE ALL RECORDS!!"q=Batch.objects.all().delete()static_path = settings.STATICFILES_DIRS[0]raw_path = os.path.join(static_path,'raw')dataset_path = os.path.join(static_path,'dataset')raw_files = fnmatch.filter(os.listdir(dataset_path), '*.jpg')for i in raw_files: _dst=os.path.join(raw_path, i ) _src=os.path.join(dataset_path,i) print "moving to: %s"%(_dst) shutil.move(src=_src, dst=_dst)
###Code
# moving from /raw/i to /dataset/j
static_path = settings.STATICFILES_DIRS[0]
raw_path = os.path.join(static_path,'raw')
dataset_path = os.path.join(static_path,'dataset')
raw_files = fnmatch.filter(os.listdir(raw_path), '*.png')
for chunk in getbatchlist(raw_files):
b=Batch()
b.save()
for i in chunk:
j=unicode(uuid4())+'.png'
print "batch: %s,src: %s, dst: %s"%(b,i,j)
Image(batch=b, src_path=j, raw_path=i).save()
_dst=os.path.join(dataset_path,j)
_src=os.path.join(raw_path,i)
shutil.move(src=_src, dst=_dst)
###Output
batch: BID000365,src: 20001309.png, dst: 92236b2b-68b8-49ad-9ab0-e1aa916fbb48.png
batch: BID000365,src: 10000658.png, dst: 80a429af-4400-4bf0-9578-eb165ec83eba.png
batch: BID000365,src: 30001999.png, dst: 12310b56-93f5-4d33-9695-bd59dc907beb.png
batch: BID000365,src: 00000256.png, dst: 71e91a98-6760-45ee-ada4-4d329b71bfb9.png
batch: BID000365,src: 40002767.png, dst: 8e1bdf5a-034e-4269-9cc6-557c258c1e54.png
batch: BID000366,src: 20001363.png, dst: 25699e2b-298e-426e-8158-cbecef851b03.png
batch: BID000366,src: 40002896.png, dst: 5a792a69-3a10-4a5c-a503-0b27c17a909a.png
batch: BID000366,src: 30001898.png, dst: e34a8216-2be4-4c9d-926b-e2defe8ae853.png
batch: BID000366,src: 20001650.png, dst: 595ed40c-121e-4d75-b2e6-c8f1b7a6d705.png
batch: BID000366,src: 40002579.png, dst: 0b329c95-e17a-48d6-9022-105ace3c66af.png
batch: BID000367,src: 20001736.png, dst: d6bb638c-8288-44bd-924a-5997fa68f7f3.png
batch: BID000367,src: 40002889.png, dst: 2c4d1992-790e-470c-97c6-a72bb4a33ea5.png
batch: BID000367,src: 20001784.png, dst: 8a5045b0-a220-451a-9329-c619419e163f.png
batch: BID000367,src: 20001281.png, dst: 23cce06b-e251-4c81-a5fd-7a673defc52d.png
batch: BID000367,src: 10000918.png, dst: 953a9460-54a7-47cc-a09d-0816c1f32762.png
batch: BID000368,src: 40002677.png, dst: 2831edc0-308a-4893-8601-ebed33771ec5.png
batch: BID000368,src: 10000597.png, dst: 4929e425-1483-4ca5-a515-9ffe64d3a77c.png
batch: BID000368,src: 40002790.png, dst: 4cd606c6-7861-4a49-b977-35b00bfd2a39.png
batch: BID000368,src: 20001723.png, dst: bb70b291-0c19-43ed-a77e-55326589405e.png
batch: BID000368,src: 20001461.png, dst: a5d2516c-b507-47a1-8e35-0228efd92d05.png
batch: BID000369,src: 30002337.png, dst: 1dc4a0c8-6f50-4e6b-b81c-1eb48ab74d4f.png
batch: BID000369,src: 30002265.png, dst: 42b0a296-46c6-4dab-8cbe-ac71dd8bdb7f.png
batch: BID000369,src: 30001872.png, dst: b958a8de-95a6-429f-ad66-bc47deadc6cb.png
batch: BID000369,src: 10001139.png, dst: 80624497-13e1-4a73-a25b-d2d142e02415.png
batch: BID000369,src: 10000643.png, dst: 7358c4bc-505e-4774-afe6-32994d809c1b.png
batch: BID000370,src: 30002358.png, dst: 166fe2d4-1354-437d-90b7-e81bfe084ac1.png
batch: BID000370,src: 30002245.png, dst: 63d032be-9d4d-4b7e-b9c4-14b250823b88.png
batch: BID000370,src: 20001328.png, dst: 311a6e09-2c69-4d8e-b6c9-f6eb2ff7f406.png
batch: BID000370,src: 20001569.png, dst: ec1fd2ad-a1cb-436b-8491-20d67a073283.png
batch: BID000370,src: 40002785.png, dst: 429b9683-9016-4275-96df-97b4775ff790.png
batch: BID000371,src: 00000218.png, dst: 9c7b4ce4-18a2-46e5-8d4c-e666bf45eee4.png
batch: BID000371,src: 10000933.png, dst: 7a1a5dae-dc90-4bfe-9602-9fee984498f1.png
batch: BID000371,src: 00000335.png, dst: c238df24-a9cc-4b97-bd01-6c1ac1840326.png
batch: BID000371,src: 30002077.png, dst: 10acb3bc-ad37-45ea-9d8a-57ad19d03513.png
batch: BID000371,src: 10001069.png, dst: fc8039be-cb76-496d-8698-bc1cd0704ef2.png
batch: BID000372,src: 40002587.png, dst: 37dacb45-fe58-4ecf-82e1-8f2cb9b2689d.png
batch: BID000372,src: 30001845.png, dst: a37444d5-1356-4c82-a7fb-d9b152305026.png
batch: BID000372,src: 10000839.png, dst: faf9cf15-d7f9-4ae6-80a6-6cea0b2e1b7b.png
batch: BID000372,src: 20001504.png, dst: a4ebd625-a694-479f-9e9e-bd04678221d9.png
batch: BID000372,src: 20001295.png, dst: dd665a73-f2cd-4bbf-b12d-a4c3095460c7.png
batch: BID000373,src: 10000592.png, dst: ad503090-8d0e-4793-8e7c-05b82061a48a.png
batch: BID000373,src: 00000059.png, dst: 8bc4de7c-5686-4a45-92e7-628059b7b433.png
batch: BID000373,src: 10001208.png, dst: 82307e37-c88b-4930-95b4-32f66c054229.png
batch: BID000373,src: 30001859.png, dst: 2ba71cc0-1b1b-4925-9086-f103e31825da.png
batch: BID000373,src: 20001401.png, dst: db04b301-bf66-4aa5-9a7d-c67c21899d6a.png
batch: BID000374,src: 20001319.png, dst: 4d5bdeda-d12d-4641-aa40-a24323db2973.png
batch: BID000374,src: 20001350.png, dst: 2961011b-5307-489b-b395-15a033ecf6c1.png
batch: BID000374,src: 20001590.png, dst: 2f352de8-7075-4c11-b7d9-905001b07dff.png
batch: BID000374,src: 30002279.png, dst: 0a56f500-7323-4eec-a173-7ed7313cc4f9.png
batch: BID000374,src: 30002460.png, dst: 0f6ccc2f-333f-44bb-b6ad-662a6baf90e6.png
batch: BID000375,src: 40002694.png, dst: e968c5f5-d02d-44a0-a9f1-5d6cc9d85c22.png
batch: BID000375,src: 10000912.png, dst: 341e5d64-a325-4b6a-83b9-7093b90197b9.png
batch: BID000375,src: 30002157.png, dst: 65254b7f-8ef0-4b52-946b-50d3f41720e3.png
batch: BID000375,src: 20001336.png, dst: 56023750-fa18-44cc-a866-62bd011d632c.png
batch: BID000375,src: 00000028.png, dst: 22cbcf02-40a4-4fd2-8fa5-eb78234afadf.png
batch: BID000376,src: 20001561.png, dst: 37029c7b-5a3e-4185-8277-fd8e07fe2353.png
batch: BID000376,src: 20001671.png, dst: 1e9881cb-50da-411c-9a13-f54f8dc4dbb4.png
batch: BID000376,src: 20001743.png, dst: 246a29a1-9890-4435-937e-1c9070363655.png
batch: BID000376,src: 10000744.png, dst: 8d98fbb4-863c-4e28-8d7d-eaab94fbba3f.png
batch: BID000376,src: 30002086.png, dst: d2bfa102-7263-4134-825b-c1ab182eeadc.png
batch: BID000377,src: 30001883.png, dst: 9c46a1db-daf2-4f1a-968b-fe30e07eb00c.png
batch: BID000377,src: 10000925.png, dst: 13415c02-732e-4088-8e90-8a9568110d15.png
batch: BID000377,src: 30002341.png, dst: 22d95dc1-3495-4bdb-82b1-7b2bc9af2d99.png
batch: BID000377,src: 20001622.png, dst: af498b85-1c59-4d88-b846-c1733c1cbb46.png
batch: BID000377,src: 00000013.png, dst: a1ed11af-2110-4357-a4a7-afbdd5fdfea2.png
batch: BID000378,src: 20001370.png, dst: 753ad744-99a8-4a54-8fcd-a85843c682b9.png
batch: BID000378,src: 40002924.png, dst: 33707828-a077-4bee-b435-fc079d7bea6f.png
batch: BID000378,src: 30002223.png, dst: 559a3f86-20a9-409c-8d03-ba8cdc297c8b.png
batch: BID000378,src: 20001450.png, dst: cc82b717-86c7-4eb8-9b71-a0cca754caa4.png
batch: BID000378,src: 20001497.png, dst: 078c62cb-af40-460d-9d9d-c59c0e6a8d12.png
batch: BID000379,src: 00000204.png, dst: 24dd812f-b916-4d0e-9411-bb6d1daf6f97.png
batch: BID000379,src: 30002095.png, dst: da21754c-694e-4006-9e40-06561102dcc0.png
batch: BID000379,src: 10000561.png, dst: ac7cf34a-61cc-4ef1-8841-41175855067b.png
batch: BID000379,src: 00000179.png, dst: d69669ba-d9b9-4a58-a9b3-13ff76c4edc6.png
batch: BID000379,src: 30002363.png, dst: d4af3975-d602-4c11-91b1-e36bd760e7cc.png
batch: BID000380,src: 00000389.png, dst: a4d0af5d-c929-4ca7-af98-adcb8d586278.png
batch: BID000380,src: 30001910.png, dst: db5dd2dc-54c2-4fab-aeaf-97ad6812d001.png
batch: BID000380,src: 20001795.png, dst: e84bc055-8270-4b30-8886-cdd4ccdf02b5.png
batch: BID000380,src: 30002024.png, dst: 88cb0cee-67f0-432e-8567-a575f7182f68.png
batch: BID000380,src: 40002764.png, dst: df01f156-f827-44a7-86db-4cc2720b2061.png
batch: BID000381,src: 00000432.png, dst: 2b693400-c2dc-45cf-8f0e-583d57629798.png
batch: BID000381,src: 10000823.png, dst: 1f009b81-4642-4d2e-814e-167e7e8a9fae.png
batch: BID000381,src: 40002667.png, dst: a363198e-028a-492b-b370-c5c3f9004a82.png
batch: BID000381,src: 40002540.png, dst: d49acb79-5b27-43c5-a5b8-d5e3c619a46d.png
batch: BID000381,src: 20001245.png, dst: 0a188ed3-6ed1-4df1-bc09-d98b753084e7.png
batch: BID000382,src: 20001825.png, dst: 21735d1c-cafb-49ac-82ec-7d25e68c2b10.png
batch: BID000382,src: 40002744.png, dst: ca7f9fc1-3578-451b-b630-628a3fb8017c.png
batch: BID000382,src: 40002652.png, dst: a878035f-c745-45a1-ac71-465ec89d734a.png
batch: BID000382,src: 40002613.png, dst: b552cb47-502b-4c17-8e12-b81387ccc4f4.png
batch: BID000382,src: 10000618.png, dst: 1b6cc51c-b9bd-4ff8-8e59-48de73fb0169.png
batch: BID000383,src: 00000130.png, dst: f37e8f8c-c66a-4f41-bd33-2d9153e35c2e.png
batch: BID000383,src: 40002761.png, dst: 22250a02-63ad-48fa-b642-c88207bf8375.png
batch: BID000383,src: 20001745.png, dst: 14459d9c-7a90-46a5-9676-c284dfb80f32.png
batch: BID000383,src: 30002200.png, dst: 70585a6f-10f6-4426-81a9-037ca3b679d8.png
batch: BID000383,src: 00000170.png, dst: afe83440-fc9c-4e24-867b-10b115edd041.png
batch: BID000384,src: 40002740.png, dst: daae5426-23a9-41ab-9de7-6aa6666ba0e9.png
batch: BID000384,src: 20001579.png, dst: c88ae841-a583-4767-900f-911690755d5a.png
batch: BID000384,src: 30001978.png, dst: a9066976-4f6a-4500-b3e6-8c3fa0ca36d8.png
batch: BID000384,src: 10000772.png, dst: 77c0fd11-1e08-4d1e-884b-fc24334db184.png
batch: BID000384,src: 30001901.png, dst: 61dd0b6a-3642-496b-936f-6a9bb7e00b71.png
|
C02/C02w04-02-explore_data.ipynb | ###Markdown
Explore and create ML datasetsIn this notebook, we will explore data corresponding to taxi rides in New York City to build a Machine Learning model in support of a fare-estimation tool. The idea is to suggest a likely fare to taxi riders so that they are not surprised, and so that they can protest if the charge is much higher than expected. Learning Objectives1. Access and explore a public BigQuery dataset on NYC Taxi Cab rides2. Visualize your dataset using the Seaborn library3. Inspect and clean-up the dataset for future ML model training4. Create a benchmark to judge future ML model performance off ofEach learning objective will correspond to a __TODO__ in this student lab notebook -- try to complete this notebook first and then review the [solution notebook](../solutions/explore_data.ipynb). Let's start with the Python imports that we need.
###Code
!sudo chown -R jupyter:jupyter /home/jupyter/training-data-analyst
from google.cloud import bigquery
import seaborn as sns
import matplotlib.pyplot as plt
import pandas as pd
import numpy as np
###Output
_____no_output_____
###Markdown
Extract sample data from BigQuery The dataset that we will use is a BigQuery public dataset. Click on the link, and look at the column names. Switch to the Details tab to verify that the number of records is one billion, and then switch to the Preview tab to look at a few rows.Let's write a SQL query to pick up interesting fields from the dataset. It's a good idea to get the timestamp in a predictable format.
###Code
%%bigquery
SELECT
FORMAT_TIMESTAMP(
"%Y-%m-%d %H:%M:%S %Z", pickup_datetime) AS pickup_datetime,
pickup_longitude, pickup_latitude, dropoff_longitude,
dropoff_latitude, passenger_count, trip_distance, tolls_amount,
fare_amount, total_amount
# TODO 1: Set correct BigQuery public dataset for nyc-tlc yellow taxi cab trips
# Tip: For projects with hyphens '-' be sure to escape with backticks ``
FROM `nyc-tlc.yellow.trips`
LIMIT 10
###Output
Query complete after 0.03s: 100%|██████████| 2/2 [00:00<00:00, 560.17query/s]
Downloading: 100%|██████████| 10/10 [00:00<00:00, 12.19rows/s]
###Markdown
Let's increase the number of records so that we can do some neat graphs. There is no guarantee about the order in which records are returned, and so no guarantee about which records get returned if we simply increase the LIMIT. To properly sample the dataset, let's use the HASH of the pickup time and return 1 in 100,000 records -- because there are 1 billion records in the data, we should get back approximately 10,000 records if we do this.We will also store the BigQuery result in a Pandas dataframe named "trips"
###Code
%%bigquery trips
SELECT
FORMAT_TIMESTAMP(
"%Y-%m-%d %H:%M:%S %Z", pickup_datetime) AS pickup_datetime,
pickup_longitude, pickup_latitude,
dropoff_longitude, dropoff_latitude,
passenger_count,
trip_distance,
tolls_amount,
fare_amount,
total_amount
FROM
`nyc-tlc.yellow.trips`
WHERE
ABS(MOD(FARM_FINGERPRINT(CAST(pickup_datetime AS STRING)), 100000)) = 1
print(len(trips))
# We can slice Pandas dataframes as if they were arrays
trips[:10]
###Output
_____no_output_____
###Markdown
Exploring data Let's explore this dataset and clean it up as necessary. We'll use the Python Seaborn package to visualize graphs and Pandas to do the slicing and filtering.
###Code
# TODO 2: Visualize your dataset using the Seaborn library.
# Plot the distance of the trip as X and the fare amount as Y.
ax = sns.regplot(x="trip_distance", y="fare_amount", fit_reg=False, ci=None, truncate=True, data=trips)
ax.figure.set_size_inches(10, 8)
###Output
_____no_output_____
###Markdown
Hmm ... do you see something wrong with the data that needs addressing?It appears that we have a lot of invalid data that is being coded as zero distance and some fare amounts that are definitely illegitimate. Let's remove them from our analysis. We can do this by modifying the BigQuery query to keep only trips longer than zero miles and fare amounts that are at least the minimum cab fare ($2.50).Note the extra WHERE clauses.
###Code
%%bigquery trips
SELECT
FORMAT_TIMESTAMP(
"%Y-%m-%d %H:%M:%S %Z", pickup_datetime) AS pickup_datetime,
pickup_longitude, pickup_latitude,
dropoff_longitude, dropoff_latitude,
passenger_count,
trip_distance,
tolls_amount,
fare_amount,
total_amount
FROM
`nyc-tlc.yellow.trips`
WHERE
ABS(MOD(FARM_FINGERPRINT(CAST(pickup_datetime AS STRING)), 100000)) = 1
# TODO 3: Filter the data to only include non-zero distance trips and fares above $2.50
AND trip_distance > 0.
AND fare_amount >= 2.5
print(len(trips))
ax = sns.regplot(
x="trip_distance", y="fare_amount",
fit_reg=False, ci=None, truncate=True, data=trips)
ax.figure.set_size_inches(10, 8)
###Output
_____no_output_____
###Markdown
What's up with the streaks around 45 dollars and 50 dollars? Those are fixed-amount rides from JFK and La Guardia airports into anywhere in Manhattan, i.e. to be expected. Let's list the data to make sure the values look reasonable.Let's also examine whether the toll amount is captured in the total amount.
###Code
tollrides = trips[trips["tolls_amount"] > 0]
tollrides[tollrides["pickup_datetime"] == "2012-02-27 09:19:10 UTC"]
notollrides = trips[trips["tolls_amount"] == 0]
notollrides[notollrides["pickup_datetime"] == "2012-02-27 09:19:10 UTC"]
###Output
_____no_output_____
###Markdown
Looking at a few samples above, it should be clear that the total amount reflects fare amount, toll and tip somewhat arbitrarily -- this is because when customers pay cash, the tip is not known. So, we'll use the sum of fare_amount + tolls_amount as what needs to be predicted. Tips are discretionary and do not have to be included in our fare estimation tool.Let's also look at the distribution of values within the columns.
###Code
trips.describe()
###Output
_____no_output_____
###Markdown
Hmm ... The min, max of longitude look strange.Finally, let's actually look at the start and end of a few of the trips.
###Code
def showrides(df, numlines):
lats = []
lons = []
for iter, row in df[:numlines].iterrows():
lons.append(row["pickup_longitude"])
lons.append(row["dropoff_longitude"])
lons.append(None)
lats.append(row["pickup_latitude"])
lats.append(row["dropoff_latitude"])
lats.append(None)
sns.set_style("darkgrid")
plt.figure(figsize=(10, 8))
plt.plot(lons, lats)
showrides(notollrides, 10)
showrides(tollrides, 10)
###Output
_____no_output_____
###Markdown
As you'd expect, rides that involve a toll are longer than the typical ride. Quality control and other preprocessing We need to do some clean-up of the data:New York city longitudes are around -74 and latitudes are around 41.We shouldn't have zero passengers.Clean up the total_amount column to reflect only fare_amount and tolls_amount, and then remove those two columns.Before the ride starts, we'll know the pickup and dropoff locations, but not the trip distance (that depends on the route taken), so remove it from the ML datasetDiscard the timestampWe could do preprocessing in BigQuery, similar to how we removed the zero-distance rides, but just to show you another option, let's do this in Python. In production, we'll have to carry out the same preprocessing on the real-time input data. This sort of preprocessing of input data is quite common in ML, especially if the quality-control is dynamic.
###Code
def preprocess(trips_in):
trips = trips_in.copy(deep=True)
trips.fare_amount = trips.fare_amount + trips.tolls_amount
del trips["tolls_amount"]
del trips["total_amount"]
del trips["trip_distance"] # we won't know this in advance!
qc = np.all([
trips["pickup_longitude"] > -78,
trips["pickup_longitude"] < -70,
trips["dropoff_longitude"] > -78,
trips["dropoff_longitude"] < -70,
trips["pickup_latitude"] > 37,
trips["pickup_latitude"] < 45,
trips["dropoff_latitude"] > 37,
trips["dropoff_latitude"] < 45,
trips["passenger_count"] > 0
], axis=0)
return trips[qc]
tripsqc = preprocess(trips)
tripsqc.describe()
###Output
_____no_output_____
###Markdown
The quality control has removed about 300 rows (11400 - 11101) or about 3% of the data. This seems reasonable.Let's move on to creating the ML datasets. Create ML datasets Let's split the QCed data randomly into training, validation and test sets.Note that this is not the entire data. We have 1 billion taxicab rides. This is just splitting the 10,000 rides to show you how it's done on smaller datasets. In reality, we'll have to do it on all 1 billion rides and this won't scale.
###Code
shuffled = tripsqc.sample(frac=1)
trainsize = int(len(shuffled["fare_amount"]) * 0.70)
validsize = int(len(shuffled["fare_amount"]) * 0.15)
df_train = shuffled.iloc[:trainsize, :]
df_valid = shuffled.iloc[trainsize:(trainsize + validsize), :]
df_test = shuffled.iloc[(trainsize + validsize):, :]
df_train.head(n=1)
df_train.describe()
df_valid.describe()
df_test.describe()
###Output
_____no_output_____
###Markdown
Let's write out the three dataframes to appropriately named csv files. We can use these csv files for local training (recall that these files represent only 1/100,000 of the full dataset) just to verify our code works, before we run it on all the data.
###Code
def to_csv(df, filename):
outdf = df.copy(deep=False)
outdf.loc[:, "key"] = np.arange(0, len(outdf)) # rownumber as key
# Reorder columns so that target is first column
cols = outdf.columns.tolist()
cols.remove("fare_amount")
cols.insert(0, "fare_amount")
print (cols) # new order of columns
outdf = outdf[cols]
outdf.to_csv(filename, header=False, index_label=False, index=False)
to_csv(df_train, "taxi-train.csv")
to_csv(df_valid, "taxi-valid.csv")
to_csv(df_test, "taxi-test.csv")
!head -10 taxi-valid.csv
###Output
5.5,2015-03-07 16:35:02 UTC,-73.99629211425781,40.7380485534668,-73.98902130126953,40.742923736572266,1,0
12.5,2013-03-27 03:35:00 UTC,-73.946246,40.800622,-73.983272,40.755498,1,1
4.9,2009-12-07 20:49:00 UTC,-73.96875,40.764292,-73.976423,40.762745,1,2
6.1,2009-05-27 20:37:00 UTC,-73.974018,40.757235,-73.980627,40.742258,1,3
5.0,2014-02-24 18:22:00 UTC,-73.967223,40.769257,-73.956733,40.775165,1,4
8.9,2011-09-21 13:45:06 UTC,-73.999776,40.738537,-74.009661,40.709277,1,5
7.5,2013-06-23 06:08:09 UTC,-74.007305,40.727548,-73.986695,40.74029,1,6
14.0,2015-04-13 10:18:42 UTC,-73.99315643310547,40.732337951660156,-73.9897689819336,40.757469177246094,1,7
5.3,2011-10-15 11:29:29 UTC,-73.967322,40.79325,-73.979722,40.776244,1,8
18.5,2015-02-23 19:51:31 UTC,-73.99144744873047,40.749961853027344,-74.01262664794922,40.71000289916992,1,9
###Markdown
Verify that datasets exist
###Code
!ls -l *.csv
###Output
-rw-r--r-- 1 jupyter jupyter 123156 Feb 5 06:44 taxi-test.csv
-rw-r--r-- 1 jupyter jupyter 579479 Feb 5 06:44 taxi-train.csv
-rw-r--r-- 1 jupyter jupyter 123124 Feb 5 06:44 taxi-valid.csv
###Markdown
We have 3 .csv files corresponding to train, valid, test. The ratio of file-sizes correspond to our split of the data.
###Code
%%bash
head taxi-train.csv
###Output
7.0,2014-05-20 23:09:00 UTC,-73.99739,40.756957,-73.980732,40.745002,2,0
9.7,2010-09-12 14:28:34 UTC,-73.983252,40.756078,-73.90924,40.765625,1,1
10.5,2012-02-21 11:53:00 UTC,-73.979562,40.751658,-74.00077,40.757603,1,2
4.5,2014-02-26 06:25:10 UTC,-73.994739,40.754633,-73.984699,40.755177,1,3
3.3,2011-02-23 16:02:05 UTC,-73.994125,40.746108,-74.00021,40.747702,2,4
9.5,2013-12-06 14:55:00 UTC,-73.99962,40.721992,-74.00925,40.720432,5,5
14.9,2011-11-16 10:18:30 UTC,-73.949011,40.77736,-73.991933,40.74759,1,6
7.0,2014-10-06 15:16:00 UTC,-73.978285,40.756372,-73.989658,40.746597,1,7
7.5,2014-05-01 23:42:21 UTC,-73.98844,40.729201,-73.972517,40.745396,1,8
4.1,2009-07-18 10:32:11 UTC,-73.980833,40.738042,-73.990306,40.730465,1,9
###Markdown
Looks good! We now have our ML datasets and are ready to train ML models, validate them and evaluate them. Benchmark Before we start building complex ML models, it is a good idea to come up with a very simple model and use that as a benchmark.My model is going to be to simply divide the mean fare_amount by the mean trip_distance to come up with a rate and use that to predict. Let's compute the RMSE of such a model.
###Code
def distance_between(lat1, lon1, lat2, lon2):
# Baversine formula to compute distance "as the crow flies".
lat1_r = np.radians(lat1)
lat2_r = np.radians(lat2)
lon_diff_r = np.radians(lon2 - lon1)
sin_prod = np.sin(lat1_r) * np.sin(lat2_r)
cos_prod = np.cos(lat1_r) * np.cos(lat2_r) * np.cos(lon_diff_r)
minimum = np.minimum(1, sin_prod + cos_prod)
dist = np.degrees(np.arccos(minimum)) * 60 * 1.515 * 1.609344
return dist
def estimate_distance(df):
return distance_between(
df["pickuplat"], df["pickuplon"], df["dropofflat"], df["dropofflon"])
def compute_rmse(actual, predicted):
return np.sqrt(np.mean((actual - predicted) ** 2))
def print_rmse(df, rate, name):
print ("{1} RMSE = {0}".format(
compute_rmse(df["fare_amount"], rate * estimate_distance(df)), name))
# TODO 4: Create a benchmark to judge future ML model performance off of
# Specify the five feature columns
FEATURES = ["pickuplat", "pickuplon", "dropofflat", "dropofflon", "passenger_count"]
# Specify the one target column for prediction
TARGET = "fare_amount"
columns = list([TARGET])
columns.append("pickup_datetime")
columns.extend(FEATURES) # in CSV, target is first column, after the features
columns.append("key")
df_train = pd.read_csv("taxi-train.csv", header=None, names=columns)
df_valid = pd.read_csv("taxi-valid.csv", header=None, names=columns)
df_test = pd.read_csv("taxi-test.csv", header=None, names=columns)
rate = df_train["fare_amount"].mean() / estimate_distance(df_train).mean()
print ("Rate = ${0}/km".format(rate))
print_rmse(df_train, rate, "Train")
print_rmse(df_valid, rate, "Valid")
print_rmse(df_test, rate, "Test")
###Output
Rate = $3.1937036935209546/km
Train RMSE = 10.047166686804264
Valid RMSE = 11.242928692253432
Test RMSE = 12.18692893119171
###Markdown
Benchmark on same datasetThe RMSE depends on the dataset, and for comparison, we have to evaluate on the same dataset each time. We'll use this query in later labs:
###Code
validation_query = """
SELECT
(tolls_amount + fare_amount) AS fare_amount,
pickup_datetime,
pickup_longitude AS pickuplon,
pickup_latitude AS pickuplat,
dropoff_longitude AS dropofflon,
dropoff_latitude AS dropofflat,
passenger_count*1.0 AS passengers,
"unused" AS key
FROM
`nyc-tlc.yellow.trips`
WHERE
ABS(MOD(FARM_FINGERPRINT(CAST(pickup_datetime AS STRING)), 10000)) = 2
AND trip_distance > 0
AND fare_amount >= 2.5
AND pickup_longitude > -78
AND pickup_longitude < -70
AND dropoff_longitude > -78
AND dropoff_longitude < -70
AND pickup_latitude > 37
AND pickup_latitude < 45
AND dropoff_latitude > 37
AND dropoff_latitude < 45
AND passenger_count > 0
"""
client = bigquery.Client()
df_valid = client.query(validation_query).to_dataframe()
print_rmse(df_valid, 2.59988, "Final Validation Set")
###Output
Final Validation Set RMSE = 8.135336354025394
|
Machine_Learning/Statistics_for_Machine_Learning/ML_1_12_Confusion_Matrix_(D).ipynb | ###Markdown
Confusion MatrixIn the field of machine learning and specifically the problem of statistical classification, a confusion matrix, also known as an error matrix.A confusion matrix is a table that is often used to describe the performance of a classification model (or “classifier”) on a set of test data for which the true values are known. It allows the visualization of the performance of an algorithm.It allows easy identification of confusion between classes e.g. one class is commonly mislabeled as the other. Most performance measures are computed from the confusion matrix.It gives us insight not only into the errors being made by a classifier but more importantly the types of errors that are being made.Here,* Class 1 : Positive* Class 2 : Negative Definition of the Terms:* Positive (P) : Observation is positive (for example: is an apple).* Negative (N) : Observation is not positive (for example: is not an apple).* True Positive (TP) : Observation is positive, and is predicted to be positive.* False Negative (FN) : Observation is positive, but is predicted negative.* True Negative (TN) : Observation is negative, and is predicted to be negative.* False Positive (FP) : Observation is negative, but is predicted positive. Classification Rate/Accuracy:Classification Rate or Accuracy is given by the relation:However, there are problems with accuracy. It assumes equal costs for both kinds of errors. A 99% accuracy can be excellent, good, mediocre, poor or terrible depending upon the problem. RecallRecall can be defined as the ratio of the total number of correctly classified positive examples divide to the total number of positive examples. High Recall indicates the class is correctly recognized (small number of FN).Recall is given by the relation: PrecisionTo get the value of precision we divide the total number of correctly classified positive examples by the total number of predicted positive examples. High Precision indicates an example labeled as positive is indeed positive (small number of FP).Precision is given by the relation: High recall, low precisionThis means that most of the positive examples are correctly recognized (low FN) but there are a lot of false positives. Low recall, high precisionThis shows that we miss a lot of positive examples (high FN) but those we predict as positive are indeed positive (low FP) F-measure Since we have two measures (Precision and Recall) it helps to have a measurement that represents both of them. We calculate an F-measure which uses Harmonic Mean in place of Arithmetic Mean as it punishes the extreme values more.The F-Measure will always be nearer to the smaller value of Precision or Recall. Let’s consider an example now, in which we have infinite data elements of class B and a single element of class A and the model is predicting class A against all the instances in the test data.
Here,
Precision : 0.0
Recall : 1.0
Now
Arithmetic mean: 0.5
Harmonic mean: 0.0
When taking the arithmetic mean, it would have 50% correct. Despite being the worst possible outcome! While taking the harmonic mean, the F-measure is 0.
Example to interpret confusion matrix:

For the simplification of the above confusion matrix i have added all the terms like TP,FP,etc and the row and column totals in the following image:

Now,
Classification Rate/Accuracy:
Accuracy = (TP + TN) / (TP + TN + FP + FN)= (100+50) /(100+5+10+50)= 0.90
Recall: Recall gives us an idea about when it’s actually yes, how often does it predict yes.
Recall=TP / (TP + FN)=100/(100+5)=0.95
Precision: Precsion tells us about when it predicts yes, how often is it correct.
Precision = TP / (TP + FP)=100/ (100+10)=0.91
F-measure:
Fmeasure=(2 $\times$ Recall $\times$ Precision)/(Recall+Presision)=
(2 $\times$ 0.95 $\times$ 0.91)/(0.91+0.95)=0.92 Creating a confusion matrix in Python Below is the implementation of the confusion matrix with the help of sklearn library.Please Note The 'acutual' and 'predicted' variables in the below code is used just for this example. You can replace the data after creating a machine learning model with the original data and results predicted by the model.
###Code
from sklearn.metrics import confusion_matrix
from sklearn.metrics import accuracy_score
from sklearn.metrics import classification_report
actual = [1, 1, 0, 1, 0, 0, 1, 0, 0, 0]
predicted = [1, 0, 0, 1, 0, 0, 1, 1, 1, 0]
results = confusion_matrix(actual, predicted)
print('Confusion Matrix :')
print(results)
print('Accuracy Score :',accuracy_score(actual, predicted))
print('Report : ')
print(classification_report(actual, predicted))
###Output
Confusion Matrix :
[[4 2]
[1 3]]
Accuracy Score : 0.7
Report :
precision recall f1-score support
0 0.80 0.67 0.73 6
1 0.60 0.75 0.67 4
accuracy 0.70 10
macro avg 0.70 0.71 0.70 10
weighted avg 0.72 0.70 0.70 10
|
WikiProject Clinical Trials snapshot 20220227.ipynb | ###Markdown
Import packages
###Code
!pip install sparqlwrapper
import pandas as pd
from SPARQLWrapper import SPARQLWrapper, JSON
def select(query, service='https://query.wikidata.org/sparql'):
sparql = SPARQLWrapper(service)
sparql.setQuery(query)
sparql.setReturnFormat(JSON)
result = sparql.query().convert()
return pd.json_normalize(result['results']['bindings'])
###Output
_____no_output_____
###Markdown
Table of contents >1 Model profiles 1.1 Clinical trials for Zika fever 1.2 Clinical trials using COVID-19 vaccine 1.3 Clinical trials at Vanderbilt University 1.4 Clinical trials with Julie McElrath as principal investigator 1.5 Clinical trials funded by Patient-Centered Outcomes Research Institute2 Topics by count of clinical trials 2.1 Medical conditions 2.2 Research interventions 2.3 Research sites 2.4 Principal investigators 2.5 Funders3 Organizational affiliations 3.1 Clinical trials with principal investigator and their affiliation 3.2 Clinical trials where principal investigator has Vanderbilt University affiliation 3.3 Chart of organizations by count of clinical trials 3.4 Clinical trials where the sponsor was Pfizer4 Researcher demographics 4.1 Count of principal investigators by gender 4.2 Clinical trials where the principal investigator is female 4.3 Principal investigators by occupation5 Scope of Wikidata's clinical trials content 5.1 List of clinical trials 5.2 Count of clinical trials 5.3 Most common properties applied to clinical trials 5.4 Count of statements in clinical trial records 5.5 Count of trial records in Wikidata per clinical trial registry> 1 Model profiles 1.1 Clinical trials for Zika fever
###Code
query_string = """
SELECT ?trial ?trialLabel WHERE {
?trial wdt:P31 wd:Q30612 .
{ ?trial wdt:P2175 wd:Q8071861. }
UNION
{ ?trial wdt:P2175 wd:Q27043680. }
SERVICE wikibase:label { bd:serviceParam wikibase:language "[AUTO_LANGUAGE],en". }
}"""
result=select(query_string)
result.to_csv("Clinical_trials_for_Zika_fever.csv")
result
###Output
_____no_output_____
###Markdown
1.2 Clinical trials using COVID-19 vaccine
###Code
query_string = """
SELECT ?trial ?trialLabel WHERE {
?trial wdt:P31 wd:Q30612 .
?trial wdt:P4844 ?intervention.
?intervention wdt:P31/wdt:P279* wd:Q87719492 .
SERVICE wikibase:label { bd:serviceParam wikibase:language "[AUTO_LANGUAGE],en". }
}"""
result=select(query_string)
result.to_csv("Clinical_trials_using_COVID-19_vaccine.csv")
result
###Output
_____no_output_____
###Markdown
1.3 Clinical trials at Vanderbilt University
###Code
query_string = """
SELECT ?trial ?trialLabel WHERE {
?trial wdt:P31 wd:Q30612 .
{ ?trial wdt:P6153 [ wdt:P749* wd:Q29052 ] .}
SERVICE wikibase:label { bd:serviceParam wikibase:language "[AUTO_LANGUAGE],en". }
}"""
result=select(query_string)
result.to_csv("Clinical_trials_at_Vanderbilt_University.csv")
result
###Output
_____no_output_____
###Markdown
1.4 Clinical trials with Julie McElrath as principal investigator
###Code
query_string = """
SELECT ?trial ?trialLabel WHERE {
?trial wdt:P31 wd:Q30612 .
{ ?trial wdt:P8329 wd:Q22006776 .}
SERVICE wikibase:label { bd:serviceParam wikibase:language "[AUTO_LANGUAGE],en". }
}"""
result=select(query_string)
result.to_csv("Clinical_trials_with_Julie_McElrath_as_principal_investigator.csv")
result
###Output
_____no_output_____
###Markdown
1.5 Clinical trials funded by Patient-Centered Outcomes Research Institute
###Code
query_string = """
SELECT ?trial ?trialLabel ?link WHERE {
?trial wdt:P31 wd:Q30612 .
{ ?trial wdt:P8324 wd:Q7144950 .}
UNION
{ ?trial wdt:P859 wd:Q7144950 .}
?trial ?link wd:Q7144950 .
SERVICE wikibase:label { bd:serviceParam wikibase:language "[AUTO_LANGUAGE],en". }
}"""
result=select(query_string)
result.to_csv("Clinical_trials_funded_by_Patient-Centered_Outcomes_Research_Institute.csv")
result
###Output
_____no_output_____
###Markdown
2 Topics by count of clinical trials 2.1 Medical conditions
###Code
query_string = """
SELECT DISTINCT ?condition ?conditionLabel (COUNT(?trial) AS ?count)
WHERE
{
?trial p:P31/ps:P31/wdt:P279* wd:Q30612.
?trial wdt:P1050 ?condition .
SERVICE wikibase:label { bd:serviceParam wikibase:language "[AUTO_LANGUAGE],en". }
}
GROUP BY ?condition ?conditionLabel ?count
ORDER BY DESC(?count)
LIMIT 100"""
result=select(query_string)
result.to_csv("Medical_conditions.csv")
result
###Output
_____no_output_____
###Markdown
2.2 Research interventions
###Code
query_string = """
SELECT DISTINCT ?intervention ?interventionLabel (COUNT(?trial) AS ?count)
WHERE
{
?trial p:P31/ps:P31/wdt:P279* wd:Q30612.
?trial wdt:P4844 ?intervention .
SERVICE wikibase:label { bd:serviceParam wikibase:language "[AUTO_LANGUAGE],en". }
}
GROUP BY ?intervention ?interventionLabel ?count
ORDER BY DESC(?count)"""
result=select(query_string)
result.to_csv("Research_interventions.csv")
result
###Output
_____no_output_____
###Markdown
2.3 Research sites
###Code
query_string = """
SELECT DISTINCT ?research_site ?research_siteLabel (COUNT(?trial) AS ?count) WHERE {
?trial wdt:P31 wd:Q30612 .
{ ?trial wdt:P6153 ?research_site . }
UNION
{ ?trial wdt:P6153 [wdt:P749 ?research_site] . }
SERVICE wikibase:label { bd:serviceParam wikibase:language "[AUTO_LANGUAGE],en". }
}
GROUP BY ?research_site ?research_siteLabel ?count
ORDER BY DESC(?count)"""
result=select(query_string)
result.to_csv("Research_sites.csv")
result
###Output
_____no_output_____
###Markdown
2.4 Principal investigators
###Code
query_string = """#defaultView:BubbleChart
SELECT (COUNT(DISTINCT ?trial) AS ?count) ?PI ?PILabel
WHERE {
?trial wdt:P31 wd:Q30612 .
{ ?trial wdt:P8329 ?PI .}
{ ?PI wdt:P108 ?org }
UNION
{ ?PI wdt:P1416 ?org }
SERVICE wikibase:label { bd:serviceParam wikibase:language "[AUTO_LANGUAGE],en". }
}
GROUP BY ?PI ?PILabel
ORDER BY DESC(?count)"""
result=select(query_string)
result.to_csv("Principal_investigators.csv")
result
###Output
_____no_output_____
###Markdown
2.5 Funders
###Code
query_string = """
SELECT DISTINCT ?funder ?funderLabel (COUNT(?trial) AS ?count)
WHERE
{
?trial p:P31/ps:P31/wdt:P279* wd:Q30612.
{ ?trial wdt:P859 ?funder .}
UNION
{ ?trial wdt:P8324 ?funder .}
SERVICE wikibase:label { bd:serviceParam wikibase:language "[AUTO_LANGUAGE],en". }
}
GROUP BY ?funder ?funderLabel ?count
ORDER BY DESC(?count)"""
result=select(query_string)
result.to_csv("Funders.csv")
result
###Output
_____no_output_____
###Markdown
3 Organizational affiliations 3.1 Clinical trials with principal investigator and their affiliation
###Code
query_string = """
SELECT ?trial ?trialLabel ?PI ?PILabel ?org ?orgLabel WHERE {
?trial wdt:P31 wd:Q30612 .
{ ?trial wdt:P8329 ?PI .}
{ ?PI wdt:P108 ?org }
UNION
{ ?PI wdt:P1416 ?org }
SERVICE wikibase:label { bd:serviceParam wikibase:language "[AUTO_LANGUAGE],en". }
}"""
result=select(query_string)
result.to_csv("Clinical_trials_with_principal_investigator_and_their_affiliation.csv")
result
###Output
_____no_output_____
###Markdown
3.2 Clinical trials where principal investigator has Vanderbilt University affiliation
###Code
query_string = """
# Q29052 is Vanderbilt University
PREFIX target: <http://www.wikidata.org/entity/Q29052>
SELECT ?trial ?trialLabel ?PI ?PILabel WHERE {
?trial wdt:P31 wd:Q30612 .
{ ?trial wdt:P8329 ?PI .}
{ ?PI wdt:P108 [ wdt:P749* target: ] }
UNION
{ ?PI wdt:P1416 [ wdt:P749* target: ] }
SERVICE wikibase:label { bd:serviceParam wikibase:language "[AUTO_LANGUAGE],en". }
}"""
result=select(query_string)
result.to_csv("Clinical_trials_where_principal_investigator_has_Vanderbilt_University_affiliation.csv")
result
###Output
_____no_output_____
###Markdown
3.3 Chart of organizations by count of clinical trials
###Code
query_string = """
#defaultView:BubbleChart
SELECT (COUNT(DISTINCT ?trial) AS ?count) ?org ?orgLabel
WHERE {
?trial wdt:P31 wd:Q30612 .
{ ?trial wdt:P8329 ?PI .}
{ ?PI wdt:P108 ?org }
UNION
{ ?PI wdt:P1416 ?org }
SERVICE wikibase:label { bd:serviceParam wikibase:language "[AUTO_LANGUAGE],en". }
}
GROUP BY ?org ?orgLabel
ORDER BY DESC(?count)"""
result=select(query_string)
result.to_csv("Chart_of_organizations_by_count_of_clinical_trials.csv")
result
###Output
_____no_output_____
###Markdown
3.4 Clinical trials where the sponsor was Pfizer
###Code
query_string = """
SELECT ?trial ?trialLabel WHERE {
?trial wdt:P31 wd:Q30612 .
{ ?trial wdt:P859 wd:Q206921 .}
UNION
{ ?trial wdt:P8324 wd:Q206921 .}
SERVICE wikibase:label { bd:serviceParam wikibase:language "[AUTO_LANGUAGE],en". }
}"""
result=select(query_string)
result.to_csv("Clinical_trials_where_the_sponsor_was_Pfizer.csv")
result
###Output
_____no_output_____
###Markdown
4 Researcher demographics 4.1 Count of principal investigators by gender
###Code
query_string = """
SELECT (COUNT(?trial) AS ?count) ?gender ?genderLabel WHERE {
?trial wdt:P31 wd:Q30612 .
?trial wdt:P8329 ?pi .
?pi wdt:P21 ?gender .
?pi wikibase:sitelinks ?sl .
SERVICE wikibase:label { bd:serviceParam wikibase:language "[AUTO_LANGUAGE],en". }
}
GROUP BY ?count ?gender ?genderLabel"""
result=select(query_string)
result.to_csv("Count_of_principal_investigators_by_gender.csv")
result
###Output
_____no_output_____
###Markdown
4.2 Clinical trials where the principal investigator is female
###Code
query_string = """
SELECT ?trial ?trialLabel ?pi ?piLabel ?sl WHERE {
?trial wdt:P31 wd:Q30612 .
?trial wdt:P8329 ?pi .
?pi wdt:P21 wd:Q6581072 .
?pi wikibase:sitelinks ?sl .
SERVICE wikibase:label { bd:serviceParam wikibase:language "[AUTO_LANGUAGE],en". }
}"""
result=select(query_string)
result.to_csv("Clinical_trials_where_the_principal_investigator_is_female.csv")
result
###Output
_____no_output_____
###Markdown
4.3 Principal investigators by occupation
###Code
query_string = """
SELECT DISTINCT ?occupation ?occupationLabel (COUNT(?trial) AS ?count) ?sl WHERE {
?trial wdt:P31 wd:Q30612 .
?trial wdt:P8329 ?pi .
?pi wdt:P106 ?occupation .
?occupation wikibase:sitelinks ?sl .
SERVICE wikibase:label { bd:serviceParam wikibase:language "[AUTO_LANGUAGE],en". }
}
GROUP BY ?count ?occupation ?occupationLabel ?sl
ORDER BY DESC(?count)"""
result=select(query_string)
result.to_csv("Principal_investigators_by_occupation.csv")
result
###Output
_____no_output_____
###Markdown
5 Scope of Wikidata's clinical trials content 5.1 List of clinical trials
###Code
query_string = """
# List of clinical trials
SELECT ?item ?nct_id ?itemLabel ?phaseLabel ?enrollment ?start_date ?primary_completion_date
WHERE
{
?item p:P31/ps:P31/wdt:P279* wd:Q30612.
?item wdt:P3098 ?nct_id .
OPTIONAL { ?item wdt:P580 ?start_date }
OPTIONAL { ?item wdt:P582 ?primary_completion_date }
OPTIONAL { ?item wdt:P6099 ?phase }
OPTIONAL { ?item wdt:P1132 ?enrollment }
SERVICE wikibase:label { bd:serviceParam wikibase:language "[AUTO_LANGUAGE],en". }
}
LIMIT 100"""
result=select(query_string)
result.to_csv("List_of_clinical_trials.csv")
result
###Output
_____no_output_____
###Markdown
5.2 Count of clinical trials
###Code
query_string = """
# Count clinical trials
SELECT (count(distinct ?item) as ?count)
WHERE {?item p:P31/ps:P31/wdt:P279* wd:Q30612
}"""
result=select(query_string)
result.to_csv("Count_of_clinical_trials.csv")
result
###Output
_____no_output_____
###Markdown
5.3 Most common properties applied to clinical trials
###Code
query_string = """
SELECT DISTINCT ?property ?propertyLabel ?count
WITH {
SELECT DISTINCT ?item WHERE {
?item wdt:P31*/wdt:P279* wd:Q30612 .
}
LIMIT 400000
} AS %items
WITH {
SELECT DISTINCT ?property (COUNT(*) AS ?count) WHERE {
INCLUDE %items.
?item ?p [ ] .
?property a wikibase:Property;
wikibase:claim ?p.
}
GROUP BY ?property
LIMIT 200
} AS %results
WHERE {
INCLUDE %results.
SERVICE wikibase:label { bd:serviceParam wikibase:language "en". }
}
ORDER BY DESC(?count)
LIMIT 200"""
result=select(query_string)
result.to_csv("Most_common_properties_applied_to_clinical_trials.csv")
result
###Output
_____no_output_____
###Markdown
5.4 Count of statements in clinical trial records
###Code
query_string = """
#defaultView:AreaChart
SELECT ?st ?ct {
{
BIND (0 AS ?ct)
BIND (0 AS ?st)
}
UNION {
SELECT ?st (COUNT(*) as ?ct)
{
?item wdt:P31*/wdt:P279* wd:Q30612 ; wikibase:statements ?st
}
GROUP BY ?st
ORDER BY ?st
}
}"""
result=select(query_string)
result.to_csv("Count_of_statements_in_clinical_trial_records.csv")
result
###Output
_____no_output_____
###Markdown
5.5 Count of trial records in Wikidata per clinical trial registry
###Code
query_string = """
SELECT DISTINCT ?registry ?registryLabel ?count
WITH {
SELECT DISTINCT ?item WHERE {
?item wdt:P31 wd:Q30612 ;
}
LIMIT 400000
} AS %items
WITH {
SELECT DISTINCT ?registry ?registryIDitem WHERE {
?registry wdt:P31 wd:Q2138567 .
?registryIDitem wdt:P1535 ?registry .
}
GROUP BY ?registry ?registryIDitem
LIMIT 100
} AS %registries
WITH {
SELECT DISTINCT ?registry (COUNT(*) AS ?count) WHERE {
INCLUDE %items.
INCLUDE %registries.
?item ?p [ ] .
?property wdt:P1629 ?registryIDitem;
wikibase:claim ?p.
?property wikibase:propertyType wikibase:ExternalId .
}
GROUP BY ?registry
LIMIT 100
} AS %results
WHERE {
INCLUDE %results.
SERVICE wikibase:label { bd:serviceParam wikibase:language "en". }
}
ORDER BY DESC(?count)
LIMIT 100"""
result=select(query_string)
result.to_csv("Count_of_trial_records_in_Wikidata_per_clinical_trial_registry.csv")
result
###Output
_____no_output_____ |
spam-codes.ipynb | ###Markdown
Spam Classification Let's Get started, I have used datasets fromUCI Spam dataset : https://www.kaggle.com/uciml/sms-spam-collection-dataset Now, here we start with spam classification so we will allotting binary values to labels so that Machine Learning model can work efficiently in predicting the results Steps taken* Load the libraries* Data Cleaning* Assigning Binary Values to Labels* Data Visualization (Part-1)* LowerCasing, Punctuation removing and Vocabulary modifications* Counting The Occurence of Words* Training, Testing Part of the model* Data Visualization (Part-2) Loading the libraries
###Code
# This Python 3 environment comes with many helpful analytics libraries installed
# It is defined by the kaggle/python Docker image: https://github.com/kaggle/docker-python
# For example, here's several helpful packages to load
import numpy as np # linear algebra
import pandas as pd # data processing, CSV file I/O (e.g. pd.read_csv)
# Input data files are available in the read-only "../input/" directory
# For example, running this (by clicking run or pressing Shift+Enter) will list all files under the input directory
from sklearn.ensemble import RandomForestClassifier, BaggingClassifier, AdaBoostClassifier
from sklearn.model_selection import train_test_split
import seaborn as sns
import matplotlib.pyplot as plt
%matplotlib inline
from sklearn.feature_extraction.text import CountVectorizer
from sklearn.metrics import accuracy_score, precision_score, f1_score, recall_score
import os
for dirname, _, filenames in os.walk('/kaggle/input'):
for filename in filenames:
print(os.path.join(dirname, filename))
# You can write up to 5GB to the current directory (/kaggle/working/) that gets preserved as output when you create a version using "Save & Run All"
# You can also write temporary files to /kaggle/temp/, but they won't be saved outside of the current session
filepath='../input/sms-spam-collection-dataset/spam.csv'
df=pd.read_csv(filepath, encoding='latin-1')
df.head()
###Output
_____no_output_____
###Markdown
Data CleaningWe, start with dropping columns with missing values
###Code
df=df.drop(['Unnamed: 2', 'Unnamed: 3', 'Unnamed: 4'],axis=1)
df=df.rename(columns={'v1':'labels','v2': 'sms'})
df.head()
###Output
_____no_output_____
###Markdown
Assigning Binary Values We fix our response values for spam and ham
###Code
df['labels']=df.labels.map({'spam':0, 'ham':1})
df.head()
df.shape
df['length']=df['sms'].apply(len)
df.head()
###Output
_____no_output_____
###Markdown
Data Visualization (Part-1)
###Code
plt.figure(figsize=(16,6))
sns.distplot(a=df['length'],kde=False)
plt.legend()
message=df[df['length']==910]['sms'].iloc[0]
message
###Output
_____no_output_____
###Markdown
LowerCasing, Punctuations and Vocab. modifications Now we will implement Bag of Words which will count the number of words based on their frequency distribution and that binary number will be fed for Machine Learning model We start with using lowercase for all the words in the above sentence
###Code
message={"""
For me the love should start
with attraction.i should feel that
I need her every time around me.she should be the first thing which comes in my thoughts.
I would start the day and end it with her.she should be there every time I dream.love will be
then when my every breath has her name.my life should happen around her.my life will be named to her.
I would cry for her.will give all my happiness and take all her sorrows.I will be ready to fight with anyone for her.
I will be in love when I will be doing the craziest things for her.love will be when I don't have to proove anyone that
my girl is the most beautiful lady on the whole planet.I will always be singing praises for her.love will be when
I start up making chicken curry and end up makiing sambar.life will be the most beautiful then.
will get every morning and thank god for the day because she is with me.I would like to say a lot..will tell later.
"""}
lower_case=[]
for i in message:
lower_case=[i.lower() for i in message]
print(lower_case)
###Output
["\nfor me the love should start \n with attraction.i should feel that \n i need her every time around me.she should be the first thing which comes in my thoughts.\n i would start the day and end it with her.she should be there every time i dream.love will be \n then when my every breath has her name.my life should happen around her.my life will be named to her.\n i would cry for her.will give all my happiness and take all her sorrows.i will be ready to fight with anyone for her.\n i will be in love when i will be doing the craziest things for her.love will be when i don't have to proove anyone that \n my girl is the most beautiful lady on the whole planet.i will always be singing praises for her.love will be when \n i start up making chicken curry and end up makiing sambar.life will be the most beautiful then.\n will get every morning and thank god for the day because she is with me.i would like to say a lot..will tell later.\n"]
###Markdown
Now we will use punctutation for sorting out the sentences
###Code
sans_punctuation = []
import string
for i in lower_case:
sans_punctuation.append(i.translate(str.maketrans('', '', string.punctuation)))
print(sans_punctuation)
###Output
['\nfor me the love should start \n with attractioni should feel that \n i need her every time around meshe should be the first thing which comes in my thoughts\n i would start the day and end it with hershe should be there every time i dreamlove will be \n then when my every breath has her namemy life should happen around hermy life will be named to her\n i would cry for herwill give all my happiness and take all her sorrowsi will be ready to fight with anyone for her\n i will be in love when i will be doing the craziest things for herlove will be when i dont have to proove anyone that \n my girl is the most beautiful lady on the whole planeti will always be singing praises for herlove will be when \n i start up making chicken curry and end up makiing sambarlife will be the most beautiful then\n will get every morning and thank god for the day because she is with mei would like to say a lotwill tell later\n']
###Markdown
**Tokenization**
###Code
preprocessed_documents = []
for i in sans_punctuation:
preprocessed_documents=[[w for w in i.split()] for i in message]
print(preprocessed_documents)
###Output
[['For', 'me', 'the', 'love', 'should', 'start', 'with', 'attraction.i', 'should', 'feel', 'that', 'I', 'need', 'her', 'every', 'time', 'around', 'me.she', 'should', 'be', 'the', 'first', 'thing', 'which', 'comes', 'in', 'my', 'thoughts.', 'I', 'would', 'start', 'the', 'day', 'and', 'end', 'it', 'with', 'her.she', 'should', 'be', 'there', 'every', 'time', 'I', 'dream.love', 'will', 'be', 'then', 'when', 'my', 'every', 'breath', 'has', 'her', 'name.my', 'life', 'should', 'happen', 'around', 'her.my', 'life', 'will', 'be', 'named', 'to', 'her.', 'I', 'would', 'cry', 'for', 'her.will', 'give', 'all', 'my', 'happiness', 'and', 'take', 'all', 'her', 'sorrows.I', 'will', 'be', 'ready', 'to', 'fight', 'with', 'anyone', 'for', 'her.', 'I', 'will', 'be', 'in', 'love', 'when', 'I', 'will', 'be', 'doing', 'the', 'craziest', 'things', 'for', 'her.love', 'will', 'be', 'when', 'I', "don't", 'have', 'to', 'proove', 'anyone', 'that', 'my', 'girl', 'is', 'the', 'most', 'beautiful', 'lady', 'on', 'the', 'whole', 'planet.I', 'will', 'always', 'be', 'singing', 'praises', 'for', 'her.love', 'will', 'be', 'when', 'I', 'start', 'up', 'making', 'chicken', 'curry', 'and', 'end', 'up', 'makiing', 'sambar.life', 'will', 'be', 'the', 'most', 'beautiful', 'then.', 'will', 'get', 'every', 'morning', 'and', 'thank', 'god', 'for', 'the', 'day', 'because', 'she', 'is', 'with', 'me.I', 'would', 'like', 'to', 'say', 'a', 'lot..will', 'tell', 'later.']]
###Markdown
Now we begin with counting the numbers as how much is their frequency
###Code
import pprint
from collections import Counter
frequency_num=[]
for i in preprocessed_documents:
frequency_count=Counter(i)
frequency_num.append(frequency_count)
pprint.pprint(frequency_num)
###Output
[Counter({'be': 11,
'will': 10,
'the': 8,
'I': 8,
'should': 5,
'for': 5,
'with': 4,
'every': 4,
'my': 4,
'and': 4,
'when': 4,
'to': 4,
'start': 3,
'her': 3,
'would': 3,
'love': 2,
'that': 2,
'time': 2,
'around': 2,
'in': 2,
'day': 2,
'end': 2,
'life': 2,
'her.': 2,
'all': 2,
'anyone': 2,
'her.love': 2,
'is': 2,
'most': 2,
'beautiful': 2,
'up': 2,
'For': 1,
'me': 1,
'attraction.i': 1,
'feel': 1,
'need': 1,
'me.she': 1,
'first': 1,
'thing': 1,
'which': 1,
'comes': 1,
'thoughts.': 1,
'it': 1,
'her.she': 1,
'there': 1,
'dream.love': 1,
'then': 1,
'breath': 1,
'has': 1,
'name.my': 1,
'happen': 1,
'her.my': 1,
'named': 1,
'cry': 1,
'her.will': 1,
'give': 1,
'happiness': 1,
'take': 1,
'sorrows.I': 1,
'ready': 1,
'fight': 1,
'doing': 1,
'craziest': 1,
'things': 1,
"don't": 1,
'have': 1,
'proove': 1,
'girl': 1,
'lady': 1,
'on': 1,
'whole': 1,
'planet.I': 1,
'always': 1,
'singing': 1,
'praises': 1,
'making': 1,
'chicken': 1,
'curry': 1,
'makiing': 1,
'sambar.life': 1,
'then.': 1,
'get': 1,
'morning': 1,
'thank': 1,
'god': 1,
'because': 1,
'she': 1,
'me.I': 1,
'like': 1,
'say': 1,
'a': 1,
'lot..will': 1,
'tell': 1,
'later.': 1})]
###Markdown
Counting The Occurence of Words Let's try the above with CountVectorizer tool
###Code
count_vector=CountVectorizer()
print(count_vector)
###Output
CountVectorizer(analyzer='word', binary=False, decode_error='strict',
dtype=<class 'numpy.int64'>, encoding='utf-8', input='content',
lowercase=True, max_df=1.0, max_features=None, min_df=1,
ngram_range=(1, 1), preprocessor=None, stop_words=None,
strip_accents=None, token_pattern='(?u)\\b\\w\\w+\\b',
tokenizer=None, vocabulary=None)
###Markdown
Now, using count_vector i have converted the words to vocabulary as well
###Code
count_vector.fit(message)
voc=count_vector.get_feature_names()
voc
###Output
_____no_output_____
###Markdown
we convert the message words to array form
###Code
doc_to_array=count_vector.transform(voc).toarray()
doc_to_array
###Output
_____no_output_____
###Markdown
Table created
###Code
frequency_matrix = pd.DataFrame(doc_to_array,
columns = count_vector.get_feature_names())
frequency_matrix
###Output
_____no_output_____
###Markdown
Training and Testing the model
###Code
from sklearn.naive_bayes import MultinomialNB
from sklearn.tree import DecisionTreeClassifier
from sklearn.neighbors import KNeighborsClassifier
X_train, X_test, y_train, y_test = train_test_split(df['sms'],df['labels'],random_state=1)
print('Number of rows in the total set: {}'.format(df.shape[0]))
print('Number of rows in the training set: {}'.format(X_train.shape[0]))
print('Number of rows in the test set: {}'.format(X_test.shape[0]))
training_data=count_vector.fit_transform(X_train)
testing_data=count_vector.transform(X_test)
mnb=MultinomialNB()
mnb.fit(training_data, y_train)
predictions=mnb.predict(testing_data)
mnb_accuracy = accuracy_score(y_test,predictions)
print('Accuracy score: ', format(accuracy_score(y_test, predictions)))
print('precision score: ', format(precision_score(y_test,predictions)))
print('recall score: ', format(recall_score(y_test,predictions)))
print('f1 score: ', format(f1_score(y_test,predictions)))
###Output
Accuracy score: 0.9856424982053122
precision score: 0.990139687756779
recall score: 0.9934047815333883
f1 score: 0.9917695473251028
###Markdown
Using Decision Trees
###Code
dtc=DecisionTreeClassifier()
dtc.fit(training_data,y_train)
predictions=dtc.predict(testing_data)
dtc_accuracy = accuracy_score(y_test,predictions)
print('Accuracy score: ', format(accuracy_score(y_test, predictions)))
print('precision score: ', format(precision_score(y_test,predictions)))
print('recall score: ', format(recall_score(y_test,predictions)))
print('f1 score: ', format(f1_score(y_test,predictions)))
###Output
Accuracy score: 0.964824120603015
precision score: 0.9841930116472546
recall score: 0.9752679307502061
f1 score: 0.9797101449275363
###Markdown
RandomForest Classifier
###Code
rfc=RandomForestClassifier()
rfc.fit(training_data,y_train)
predictions=rfc.predict(testing_data)
rfc_accuracy = accuracy_score(y_test,predictions)
print('Accuracy score: ', format(accuracy_score(y_test,predictions)))
print('precision score: ', format(precision_score(y_test,predictions)))
print('recall score: ', format(recall_score(y_test,predictions)))
print('f1 score: ', format(f1_score(y_test,predictions)))
###Output
Accuracy score: 0.9842067480258435
precision score: 0.983753046303818
recall score: 0.998351195383347
f1 score: 0.9909983633387889
###Markdown
KNN
###Code
knn=KNeighborsClassifier()
knn.fit(training_data, y_train)
predictions=knn.predict(testing_data)
knn_accuracy = accuracy_score(y_test,predictions)
print('Accuracy score: ', format(accuracy_score(y_test,predictions)))
print('precision score: ', format(precision_score(y_test,predictions)))
print('recall score: ', format(recall_score(y_test,predictions)))
print('f1 score: ', format(f1_score(y_test,predictions)))
###Output
Accuracy score: 0.9217516152189519
precision score: 0.9175491679273827
recall score: 1.0
f1 score: 0.9570019723865877
###Markdown
Bagging Classifer and AdaBoost
###Code
bgc=BaggingClassifier()
bgc.fit(training_data, y_train)
predictions=bgc.predict(testing_data)
bgc_accuracy = accuracy_score(y_test,predictions)
print('Accuracy score: ', format(accuracy_score(y_test,predictions)))
print('precision score: ', format(precision_score(y_test,predictions)))
print('recall score: ', format(recall_score(y_test,predictions)))
print('f1 score: ', format(f1_score(y_test,predictions)))
#AdaBoost
adb=AdaBoostClassifier()
adb.fit(training_data, y_train)
predictions=adb.predict(testing_data)
adb_accuracy = accuracy_score(y_test,predictions)
print('Accuracy score: ', format(accuracy_score(y_test,predictions)))
print('precision score: ', format(precision_score(y_test,predictions)))
print('recall score: ', format(recall_score(y_test,predictions)))
print('f1 score: ', format(f1_score(y_test,predictions)))
###Output
Accuracy score: 0.9763101220387652
precision score: 0.9796747967479674
recall score: 0.9934047815333883
f1 score: 0.9864920180106427
###Markdown
Data Visualization (Part-2) **Accuracy Plots estimations **
###Code
clf=(mnb_accuracy,dtc_accuracy,rfc_accuracy,knn_accuracy,bgc_accuracy,adb_accuracy)
plt.figure(figsize=(16,6))
sns.distplot(a=clf, hist=True)
plt.xlabel('Accuracy scores')
plt.title('Accuracy comparison')
plt.legend()
###Output
_____no_output_____
###Markdown
Bar plot for all model accuracies
###Code
sns.barplot(data=clf)
plt.title('Accuracy estimates')
plt.legend()
###Output
_____no_output_____ |
Recommender-Source-Code/JupyterNotebook/notebooks/Columnar_Data.ipynb | ###Markdown
In this Tutorial we will explore how to work with columnar data in HoloViews. Columnar data has a fixed list of column headings, with values stored in an arbitrarily long list of rows. Spreadsheets, relational databases, CSV files, and many other typical data sources fit naturally into this format. HoloViews defines an extensible system of interfaces to load, manipulate, and visualize this kind of data, as well as allowing conversion of any of the non-columnar data types into columnar data for analysis or data interchange.By default HoloViews will use one of three storage formats for columnar data:* A pure Python dictionary containing each column.* A purely NumPy-based format for numeric data.* Pandas DataFrames
###Code
import numpy as np
import pandas as pd
import holoviews as hv
from IPython.display import HTML
hv.notebook_extension()
###Output
_____no_output_____
###Markdown
Simple Dataset Usually when working with data we have one or more independent variables, taking the form of categories, labels, discrete sample coordinates, or bins. These variables are what we refer to as key dimensions (or ``kdims`` for short) in HoloViews. The observer or dependent variables, on the other hand, are referred to as value dimensions (``vdims``), and are ordinarily measured or calculated given the independent variables. The simplest useful form of a Dataset object is therefore a column 'x' and a column 'y' corresponding to the key dimensions and value dimensions respectively. An obvious visual representation of this data is a Table:
###Code
xs = range(10)
ys = np.exp(xs)
table = hv.Table((xs, ys), kdims=['x'], vdims=['y'])
table
###Output
_____no_output_____
###Markdown
However, this data has many more meaningful visual representations, and therefore the first important concept is that Dataset objects are interchangeable as long as their dimensionality allows it, meaning that you can easily create the different objects from the same data (and cast between the objects once created):
###Code
hv.Scatter(table) + hv.Curve(table) + hv.Bars(table)
###Output
_____no_output_____
###Markdown
Each of these three plots uses the same data, but represents a different assumption about the semantic meaning of that data -- the Scatter plot is appropriate if that data consists of independent samples, the Curve plot is appropriate for samples chosen from an underlying smooth function, and the Bars plot is appropriate for independent categories of data. Since all these plots have the same dimensionality, they can easily be converted to each other, but there is normally only one of these representations that is semantically appropriate for the underlying data. For this particular data, the semantically appropriate choice is Curve, since the *y* values are samples from the continuous function ``exp``.As a guide to which Elements can be converted to each other, those of the same dimensionality here should be interchangeable, because of the underlying similarity of their columnar representation:* 0D: BoxWhisker, Spikes, Distribution*, * 1D: Scatter, Curve, ErrorBars, Spread, Bars, BoxWhisker, Regression** 2D: Points, HeatMap, Bars, BoxWhisker, Bivariate** 3D: Scatter3D, Trisurface, VectorField, BoxWhisker, Bars\* - requires SeabornThis categorization is based only on the ``kdims``, which define the space in which the data has been sampled or defined. An Element can also have any number of value dimensions (``vdims``), which may be mapped onto various attributes of a plot such as the color, size, and orientation of the plotted items. For a reference of how to use these various Element types, see the [Elements Tutorial](Elements.ipynb). Data types and ConstructorsAs discussed above, Dataset provide an extensible interface to store and operate on data in different formats. All interfaces support a number of standard constructors. Storage formats Dataset types can be constructed using one of three supported formats, (a) a dictionary of columns, (b) an NxD array with N rows and D columns, or (c) pandas dataframes:
###Code
print(hv.Scatter({'x': xs, 'y': ys}) +
hv.Scatter(np.column_stack([xs, ys])) +
hv.Scatter(pd.DataFrame({'x': xs, 'y': ys})))
###Output
_____no_output_____
###Markdown
LiteralsIn addition to the main storage formats, Dataset Elements support construction from three Python literal formats: (a) An iterator of y-values, (b) a tuple of columns, and (c) an iterator of row tuples.
###Code
print(hv.Scatter(ys) + hv.Scatter((xs, ys)) + hv.Scatter(zip(xs, ys)))
###Output
_____no_output_____
###Markdown
For these inputs, the data will need to be copied to a new data structure, having one of the three storage formats above. By default Dataset will try to construct a simple array, falling back to either pandas dataframes (if available) or the dictionary-based format if the data is not purely numeric. Additionally, the interfaces will try to maintain the provided data's type, so numpy arrays and pandas DataFrames will therefore always be parsed by the array and dataframe interfaces first respectively.
###Code
df = pd.DataFrame({'x': xs, 'y': ys, 'z': ys*2})
print(type(hv.Scatter(df).data))
###Output
_____no_output_____
###Markdown
Dataset will attempt to parse the supplied data, falling back to each consecutive interface if the previous could not interpret the data. The default list of fallbacks and simultaneously the list of allowed datatypes is:
###Code
hv.Dataset.datatype
###Output
_____no_output_____
###Markdown
Note these include grid based datatypes, which are not covered in this tutorial. To select a particular storage format explicitly, supply one or more allowed datatypes:
###Code
print(type(hv.Scatter((xs, ys), datatype=['array']).data))
print(type(hv.Scatter((xs, ys), datatype=['dictionary']).data))
print(type(hv.Scatter((xs, ys), datatype=['dataframe']).data))
###Output
_____no_output_____
###Markdown
Sharing Data Since the formats with labelled columns do not require any specific order, each Element can effectively become a view into a single set of data. By specifying different key and value dimensions, many Elements can show different values, while sharing the same underlying data source.
###Code
overlay = hv.Scatter(df, kdims='x', vdims='y') * hv.Scatter(df, kdims='x', vdims='z')
overlay
###Output
_____no_output_____
###Markdown
We can quickly confirm that the data is actually shared:
###Code
overlay.Scatter.I.data is overlay.Scatter.II.data
###Output
_____no_output_____
###Markdown
For columnar data, this approach is much more efficient than creating copies of the data for each Element, and allows for some advanced features like linked brushing in the [Bokeh backend](Bokeh_Backend.ipynb). Converting to raw data Column types make it easy to export the data to the three basic formats: arrays, dataframes, and a dictionary of columns. Array
###Code
table.array()
###Output
_____no_output_____
###Markdown
Pandas DataFrame
###Code
HTML(table.dframe().head().to_html())
###Output
_____no_output_____
###Markdown
Dataset dictionary
###Code
table.columns()
###Output
_____no_output_____
###Markdown
Creating tabular data from Elements using the .table and .dframe methodsIf you have data in some other HoloViews element and would like to use the columnar data features, you can easily tabularize any of the core Element types into a ``Table`` Element, using the ``.table()`` method. Similarly, the ``.dframe()`` method will convert an Element into a pandas DataFrame. These methods are very useful if you want to then transform the data into a different Element type, or to perform different types of analysis. Tabularizing simple ElementsFor a simple example, we can create a ``Curve`` of an exponential function and convert it to a ``Table`` with the ``.table`` method, with the same result as creating the Table directly from the data as done earlier on this Tutorial:
###Code
xs = np.arange(10)
curve = hv.Curve(zip(xs, np.exp(xs)))
curve * hv.Scatter(zip(xs, curve)) + curve.table()
###Output
_____no_output_____
###Markdown
Similarly, we can get a pandas dataframe of the Curve using ``curve.dframe()``. Here we wrap that call as raw HTML to allow automated testing of this notebook, but just calling ``curve.dframe()`` would give the same result visually:
###Code
HTML(curve.dframe().to_html())
###Output
_____no_output_____
###Markdown
Although 2D image-like objects are *not* inherently well suited to a flat columnar representation, serializing them by converting to tabular data is a good way to reveal the differences between Image and Raster elements. Rasters are a very simple type of element, using array-like integer indexing of rows and columns from their top-left corner as in computer graphics applications. Conversely, Image elements are a higher-level abstraction that provides a general-purpose continuous Cartesian coordinate system, with x and y increasing to the right and upwards as in mathematical applications, and each point interpreted as a sample representing the pixel in which it is located (and thus centered within that pixel). Given the same data, the ``.table()`` representation will show how the data is being interpreted (and accessed) differently in the two cases (as explained in detail in the [Continuous Coordinates Tutorial](Continuous_Coordinates.ipynb)):
###Code
%%opts Points (s=200) [size_index=None]
extents = (-1.6,-2.7,2.0,3)
np.random.seed(42)
mat = np.random.rand(3, 3)
img = hv.Image(mat, bounds=extents)
raster = hv.Raster(mat)
img * hv.Points(img) + img.table() + \
raster * hv.Points(raster) + raster.table()
###Output
_____no_output_____
###Markdown
Tabularizing space containersEven deeply nested objects can be deconstructed in this way, serializing them to make it easier to get your raw data out of a collection of specialized Element types. Let's say we want to make multiple observations of a noisy signal. We can collect the data into a HoloMap to visualize it and then call ``.table()`` to get a columnar object where we can perform operations or transform it to other Element types. Deconstructing nested data in this way only works if the data is homogenous. In practical terms, the requirement is that your data structure contains Elements (of any types) in these Container types: NdLayout, GridSpace, HoloMap, and NdOverlay, with all dimensions consistent throughout (so that they can all fit into the same set of columns).Let's now go back to the Image example. We will now collect a number of observations of some noisy data into a HoloMap and display it:
###Code
obs_hmap = hv.HoloMap({i: hv.Image(np.random.randn(10, 10), bounds=(0,0,3,3))
for i in range(3)}, key_dimensions=['Observation'])
obs_hmap
###Output
_____no_output_____
###Markdown
Now we can serialize this data just as before, where this time we get a four-column (4D) table. The key dimensions of both the HoloMap and the Images, as well as the z-values of each Image, are all merged into a single table. We can visualize the samples we have collected by converting it to a Scatter3D object.
###Code
%%opts Layout [fig_size=150] Scatter3D [color_index=3 size_index=None] (cmap='hot' edgecolor='k' s=50)
obs_hmap.table().to.scatter3d() + obs_hmap.table()
###Output
_____no_output_____
###Markdown
Here the `z` dimension is shown by color, as in the original images, and the other three dimensions determine where the datapoint is shown in 3D. This way of deconstructing will work for any data structure that satisfies the conditions described above, no matter how nested. If we vary the amount of noise while continuing to performing multiple observations, we can create an ``NdLayout`` of HoloMaps, one for each level of noise, and animated by the observation number.
###Code
from itertools import product
extents = (0,0,3,3)
error_hmap = hv.HoloMap({(i, j): hv.Image(j*np.random.randn(3, 3), bounds=extents)
for i, j in product(range(3), np.linspace(0, 1, 3))},
key_dimensions=['Observation', 'noise'])
noise_layout = error_hmap.layout('noise')
noise_layout
###Output
_____no_output_____
###Markdown
And again, we can easily convert the object to a ``Table``:
###Code
%%opts Table [fig_size=150]
noise_layout.table()
###Output
_____no_output_____
###Markdown
Applying operations to the data Sorting by columns Once data is in columnar form, it is simple to apply a variety of operations. For instance, Dataset can be sorted by their dimensions using the ``.sort()`` method. By default, this method will sort by the key dimensions, but any other dimension(s) can be supplied to specify sorting along any other dimensions:
###Code
bars = hv.Bars((['C', 'A', 'B', 'D'], [2, 7, 3, 4]))
bars + bars.sort() + bars.sort(['y'])
###Output
_____no_output_____
###Markdown
Working with categorical or grouped data Data is often grouped in various ways, and the Dataset interface provides various means to easily compare between groups and apply statistical aggregates. We'll start by generating some synthetic data with two groups along the x-axis and 4 groups along the y axis.
###Code
n = np.arange(1000)
xs = np.repeat(range(2), 500)
ys = n%4
zs = np.random.randn(1000)
table = hv.Table((xs, ys, zs), kdims=['x', 'y'], vdims=['z'])
table
###Output
_____no_output_____
###Markdown
Since there are repeat observations of the same x- and y-values, we have to reduce the data before we display it or else use a datatype that supports plotting distributions in this way. The ``BoxWhisker`` type allows doing exactly that:
###Code
%%opts BoxWhisker [aspect=2 fig_size=200 bgcolor='w']
hv.BoxWhisker(table)
###Output
_____no_output_____
###Markdown
Aggregating/Reducing dimensions Most types require the data to be non-duplicated before being displayed. For this purpose, HoloViews makes it easy to ``aggregate`` and ``reduce`` the data. These two operations are simple inverses of each other--aggregate computes a statistic for each group in the supplied dimensions, while reduce combines all the groups except the supplied dimensions. Supplying only a function and no dimensions will simply aggregate or reduce all available key dimensions.
###Code
%%opts Bars [show_legend=False] {+axiswise}
hv.Bars(table).aggregate(function=np.mean) + hv.Bars(table).reduce(x=np.mean)
###Output
_____no_output_____
###Markdown
(**A**) aggregates over both the x and y dimension, computing the mean for each x/y group, while (**B**) reduces the x dimension leaving just the mean for each group along y. Collapsing multiple Dataset Elements When multiple observations are broken out into a HoloMap they can easily be combined using the ``collapse`` method. Here we create a number of Curves with increasingly larger y-values. By collapsing them with a ``function`` and a ``spreadfn`` we can compute the mean curve with a confidence interval. We then simply cast the collapsed ``Curve`` to a ``Spread`` and ``Curve`` Element to visualize them.
###Code
hmap = hv.HoloMap({i: hv.Curve(np.arange(10)*i) for i in range(10)})
collapsed = hmap.collapse(function=np.mean, spreadfn=np.std)
hv.Spread(collapsed) * hv.Curve(collapsed) + collapsed.table()
###Output
_____no_output_____
###Markdown
Working with complex dataIn the last section we only scratched the surface of what the Dataset interface can do. When it really comes into its own is when working with high-dimensional datasets. As an illustration, we'll load a dataset of some macro-economic indicators for OECD countries from 1964-1990, cached on the HoloViews website.
###Code
macro_df = pd.read_csv('http://assets.holoviews.org/macro.csv', '\t')
HTML(macro_df.head().to_html())
###Output
_____no_output_____
###Markdown
As we can see the data has abbreviated the names of the columns, which is convenient when referring to the variables but is often not what's desired when assigning axis labels, generating widgets, or adding titles.HoloViews dimensions provide a way to alias the variable names so you can continue to refer to the data by their short convenient ``name`` but can also provide a more descriptive ``label``. These can be declared explicitly when creating a Dimension but the most convenient way of specifying aliases is as a tuple where the first item is the ``name`` and the second the ``label``. Here will declare a list of key dimensions (i.e. the variables the data is indexed by) and a separate list of value dimensions (i.e. the actual observations), which we will use later when declaring a HoloViews object from our data.
###Code
key_dimensions = [('year', 'Year'), ('country', 'Country')]
value_dimensions = [('unem', 'Unemployment'), ('capmob', 'Capital Mobility'),
('gdp', 'GDP Growth'), ('trade', 'Trade')]
###Output
_____no_output_____
###Markdown
We'll also take this opportunity to set default options for all the following plots.
###Code
%output dpi=100
options = hv.Store.options()
opts = hv.Options('plot', aspect=2, fig_size=250, show_frame=False, show_grid=True, legend_position='right')
options.NdOverlay = opts
options.Overlay = opts
###Output
_____no_output_____
###Markdown
Loading the dataAs we saw above, we can supply a dataframe to any Dataset type. When dealing with so many dimensions it would be cumbersome to supply all the dimensions explicitly, but luckily Dataset can easily infer the dimensions from the dataframe itself. We simply supply the ``kdims``, and it will infer that all other numeric dimensions should be treated as value dimensions (``vdims``).
###Code
macro = hv.Table(macro_df, kdims=key_dimensions, vdims=value_dimensions)
###Output
_____no_output_____
###Markdown
To get an overview of the data we'll quickly sort it and then view the data for one year.
###Code
%%opts Table [aspect=1.5 fig_size=300]
macro = macro.sort()
macro[1988]
###Output
_____no_output_____
###Markdown
Most of the examples above focus on converting a Table to simple Element types, but HoloViews also provides powerful container objects to explore high-dimensional data, such as [HoloMap](Containers.ipynbHoloMap), [NdOverlay](Containers.ipynbNdOverlay), [NdLayout](Containers.ipynbNdLayout), and [GridSpace](Containers.ipynbLayout). HoloMaps work as a useful interchange format from which you can conveniently convert to the other container types using its ``.overlay()``, ``.layout()``, and ``.grid()`` methods. This way we can easily create an overlay of GDP Growth curves by year for each country. Here ``Year`` is a key dimension and ``GDP Growth`` a value dimension. We are then left with the ``Country`` dimension, which we can overlay using the ``.overlay()`` method.
###Code
%%opts Curve (color=Palette('Set3'))
gdp_curves = macro.to.curve('Year', 'GDP Growth')
gdp_curves.overlay('Country')
###Output
_____no_output_____
###Markdown
Now that we've extracted the ``gdp_curves``, we can apply some operations to them. As in the simpler example above we will ``collapse`` the HoloMap of Curves using a number of functions to visualize the distribution of GDP Growth rates over time. First we find the mean curve with ``np.std`` as the ``spreadfn`` and cast the result to a ``Spread`` type, then we compute the min, mean and max curve in the same way and put them all inside an Overlay.
###Code
%%opts Overlay [bgcolor='w' legend_position='top_right'] Curve (color='k' linewidth=1) Spread (facecolor='gray' alpha=0.2)
hv.Spread(gdp_curves.collapse('Country', np.mean, np.std), label='std') *\
hv.Overlay([gdp_curves.collapse('Country', fn).relabel(name)(style=dict(linestyle=ls))
for name, fn, ls in [('max', np.max, '--'), ('mean', np.mean, '-'), ('min', np.min, '--')]])
###Output
_____no_output_____
###Markdown
Many HoloViews Element types support multiple ``kdims``, including ``HeatMap``, ``Points``, ``Scatter``, ``Scatter3D``, and ``Bars``. ``Bars`` in particular allows you to lay out your data in groups, categories and stacks. By supplying the index of that dimension as a plotting option you can choose to lay out your data as groups of bars, categories in each group, and stacks. Here we choose to lay out the trade surplus of each country with groups for each year, no categories, and stacked by country. Finally, we choose to color the ``Bars`` for each item in the stack.
###Code
%opts Bars [bgcolor='w' aspect=3 figure_size=450 show_frame=False]
%%opts Bars [category_index=2 stack_index=0 group_index=1 legend_position='top' legend_cols=7 color_by=['stack']] (color=Palette('Dark2'))
macro.to.bars(['Country', 'Year'], 'Trade', [])
###Output
_____no_output_____
###Markdown
This plot contains a lot of data, and so it's probably a good idea to focus on specific aspects of it, telling a simpler story about them. For instance, using the .select method we can then customize the palettes (e.g. to use consistent colors per country across multiple analyses).Palettes can customized by selecting only a subrange of the underlying cmap to draw the colors from. The Palette draws samples from the colormap using the supplied ``sample_fn``, which by default just draws linear samples but may be overriden with any function that draws samples in the supplied ranges. By slicing the ``Set1`` colormap we draw colors only from the upper half of the palette and then reverse it.
###Code
%%opts Bars [padding=0.02 color_by=['group']] (alpha=0.6, color=Palette('Set1', reverse=True)[0.:.2])
countries = {'Belgium', 'Netherlands', 'Sweden', 'Norway'}
macro.to.bars(['Country', 'Year'], 'Unemployment').select(Year=(1978, 1985), Country=countries)
###Output
_____no_output_____
###Markdown
Many HoloViews Elements support multiple key and value dimensions. A HeatMap is indexed by two kdims, so we can visualize each of the economic indicators by year and country in a Layout. Layouts are useful for heterogeneous data you want to lay out next to each other.Before we display the Layout let's apply some styling; we'll suppress the value labels applied to a HeatMap by default and substitute it for a colorbar. Additionally we up the number of xticks that are drawn and rotate them by 90 degrees to avoid overlapping. Flipping the y-axis ensures that the countries appear in alphabetical order. Finally we reduce some of the margins of the Layout and increase the size.
###Code
%opts HeatMap [show_values=False xticks=40 xrotation=90 aspect=1.2 invert_yaxis=True colorbar=True]
%%opts Layout [aspect_weight=1 fig_size=150 sublabel_position=(-0.2, 1.)]
hv.Layout([macro.to.heatmap(['Year', 'Country'], value)
for value in macro.data.columns[2:]]).cols(2)
###Output
_____no_output_____
###Markdown
Another way of combining heterogeneous data dimensions is to map them to a multi-dimensional plot type. Scatter Elements, for example, support multiple ``vdims``, which may be mapped onto the color and size of the drawn points in addition to the y-axis position. As for the Curves above we supply 'Year' as the sole key dimension and rely on the Table to automatically convert the Country to a map dimension, which we'll overlay. However this time we select both GDP Growth and Unemployment, to be plotted as points. To get a sensible chart, we adjust the scaling_factor for the points to get a reasonable distribution in sizes and apply a categorical Palette so we can distinguish each country.
###Code
%%opts Scatter [scaling_method='width' scaling_factor=2 size_index=2] (color=Palette('Set3') edgecolors='k')
gdp_unem_scatter = macro.to.scatter('Year', ['GDP Growth', 'Unemployment'])
gdp_unem_scatter.overlay('Country')
###Output
_____no_output_____
###Markdown
In this way we can plot any dimension against any other dimension, very easily allowing us to iterate through different ways of revealing relationships in the dataset.
###Code
%%opts NdOverlay [legend_cols=2] Scatter [size_index=1] (color=Palette('Blues'))
macro.to.scatter('GDP Growth', 'Unemployment', ['Year']).overlay()
###Output
_____no_output_____
###Markdown
This view, for example, immediately highlights the high unemployment rates of the 1980s. Since all HoloViews Elements are composable, we can generate complex figures just by applying the * operator. We'll simply reuse the GDP curves we generated earlier, combine them with the scatter points (which indicate the unemployment rate by size) and annotate the data with some descriptions of what happened economically in these years.
###Code
%%opts Curve (color='k') Scatter [color_index=2 size_index=2 scaling_factor=1.4] (cmap='Blues' edgecolors='k')
macro_overlay = gdp_curves * gdp_unem_scatter
annotations = hv.Arrow(1973, 8, 'Oil Crisis', 'v') * hv.Arrow(1975, 6, 'Stagflation', 'v') *\
hv.Arrow(1979, 8, 'Energy Crisis', 'v') * hv.Arrow(1981.9, 5, 'Early Eighties\n Recession', 'v')
macro_overlay * annotations
###Output
_____no_output_____
###Markdown
Since we didn't map the country to some other container type, we get a widget allowing us to view the plot separately for each country, reducing the forest of curves we encountered before to manageable chunks. While looking at the plots individually like this allows us to study trends for each country, we may want to lay out a subset of the countries side by side, e.g. for non-interactive publications. We can easily achieve this by selecting the countries we want to view and and then applying the ``.layout`` method. We'll also want to restore the square aspect ratio so the plots compose nicely.
###Code
%%opts NdLayout [figure_size=100] Overlay [aspect=1] Scatter [color_index=2] (cmap='Reds')
countries = {'United States', 'Canada', 'United Kingdom'}
(gdp_curves * gdp_unem_scatter).select(Country=countries).layout('Country')
###Output
_____no_output_____
###Markdown
Finally, let's combine some plots for each country into a Layout, giving us a quick overview of each economic indicator for each country:
###Code
%%opts Scatter [color_index=2] (cmap='Reds') Overlay [aspect=1]
(macro_overlay.relabel('GDP Growth', depth=1) +\
macro.to.curve('Year', 'Unemployment', ['Country'], group='Unemployment',) +\
macro.to.curve('Year', 'Trade', ['Country'], group='Trade') +\
macro.to.scatter('GDP Growth', 'Unemployment', ['Country'])).cols(2)
###Output
_____no_output_____ |
ComplementaryScripts/1. MappingMNXtoBIGG.ipynb | ###Markdown
General Import of the Model and Modules.
###Code
from pandas import read_csv
import pandas as pd
import os
import re
import math
import cameo as cameo
from cameo.flux_analysis.simulation import pfba
import cobra.test
relative_directory = os.getcwd()
filename = relative_directory + '/Reconstructions/BMID000000141026-M3.xml'
cameo_model = cameo.load_model(filename)
###Output
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_h_c appears as a reactant and product MNXR37123_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_MNXM54123_c appears as a reactant and product MNXR59222_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_h_c appears as a reactant and product MNXR55853_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_MNXM39835_c appears as a reactant and product MNXR59152_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_MNXM39835_c appears as a reactant and product MNXR59150_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_dna_c appears as a reactant and product MNXR14582_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_h_c appears as a reactant and product MNXR54990_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_h_c appears as a reactant and product MNXR55609_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_MNXM39835_c appears as a reactant and product MNXR59154_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_MNXM39835_c appears as a reactant and product MNXR59156_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_dna_c appears as a reactant and product MNXR14583_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_h_c appears as a reactant and product MNXR59149_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_MNXM39835_c appears as a reactant and product MNXR59153_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_MNXM39835_c appears as a reactant and product MNXR59158_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_MNXM39835_c appears as a reactant and product MNXR59157_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_MNXM39835_c appears as a reactant and product MNXR59155_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_dna_c appears as a reactant and product MNXR14584_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_dna_c appears as a reactant and product MNXR14585_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_h_c appears as a reactant and product MNXR60401_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_pepd_c appears as a reactant and product MNXR59113_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_h_c appears as a reactant and product MNXR3912_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_h_c appears as a reactant and product MNXR15372_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_h_c appears as a reactant and product MNXR55908_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_h_c appears as a reactant and product MNXR15847_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_h_c appears as a reactant and product MNXR61122_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_MNXM90460_c appears as a reactant and product MNXR35780_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_MNXM60163_c appears as a reactant and product MNXR59368_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_h_c appears as a reactant and product MNXR5200_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_h_c appears as a reactant and product MNXR31449_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_h_c appears as a reactant and product MNXR5890_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_h_c appears as a reactant and product MNXR61047_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_h_c appears as a reactant and product MNXR61048_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_h_c appears as a reactant and product MNXR55430_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_h_c appears as a reactant and product MNXR55443_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_h_c appears as a reactant and product MNXR55878_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_h_c appears as a reactant and product MNXR4584_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_MNXM13005_c appears as a reactant and product MNXR36005_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_h_c appears as a reactant and product MNXR4511_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_h_c appears as a reactant and product MNXR19140_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_h_c appears as a reactant and product MNXR60183_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_h_c appears as a reactant and product MNXR3483_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_h_c appears as a reactant and product MNXR55693_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_MNXM1682_c appears as a reactant and product MNXR14977_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_h_c appears as a reactant and product MNXR14134_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_h_c appears as a reactant and product MNXR6366_c
/Users/clie/Dev/cobrapy/cobra/io/sbml.py:205: UserWarning:
M_MNXM1682_c appears as a reactant and product MNXR14975_c
###Markdown
Metabolites Import of the old (ver 1.0) and most recent (ver 2.0) dumps of the MetaNetX DBExtract Metabolite IDs from the model and parse them in the most recent and old MNX dumps (chem_prop_2.tsv.txt and chem_prop_1.tsv.txt)
###Code
chem_prop_MNX_1 = read_csv('/Users/clie/Desktop/EFPro2/Calculation Results/MetanetX Mapping/chem_prop_1.tsv.txt', delimiter='\t',skiprows=124)
chem_prop_MNX_1_reordered = chem_prop_MNX_1.set_index('#MNX_ID')
chem_prop_MNX_1_dict = chem_prop_MNX_1_reordered.to_dict('index')
chem_prop_MNX_2 = read_csv('/Users/clie/Desktop/EFPro2/Calculation Results/MetanetX Mapping/chem_prop_2.tsv.txt', delimiter='\t',skiprows=126)
chem_prop_MNX_2_reordered = chem_prop_MNX_2.set_index('#MNX_ID')
chem_prop_MNX_2_dict = chem_prop_MNX_2_reordered.to_dict('index')
# MNX ID as keys
# chem_xref_MNX_1 = read_csv('/Users/clie/Desktop/EFPro2/Calculation Results/MetanetX Mapping/chem_xref_1_alt.tsv.txt', delimiter='\t')
# df_1 = chem_xref_MNX_1.loc[:,'XRef':'MNX_ID']
# groups_1 = df_1.groupby(['MNX_ID','XRef'])
# df_1 = groups_1.apply(lambda x:list(x['XRef_ID']))
# df_1 =df_1.unstack('XRef')
# chem_xref_MNX_1_dict = df_1.to_dict('index')
chem_xref_MNX_2 = read_csv('/Users/clie/Desktop/EFPro2/Calculation Results/MetanetX Mapping/chem_xref_2_alt.tsv.txt', delimiter='\t')
df_2 = chem_xref_MNX_2.loc[:,'XRef':'MNX_ID']
groups = df_2.groupby(['MNX_ID','XRef'])
df_2 = groups.apply(lambda x:list(x['XRef_ID']))
df_2 =df_2.unstack('XRef')
chem_xref_MNX_2_dict = df_2.to_dict('index')
# KEGG ID as keys
chem_xref_MNX_Kegg = read_csv('/Users/clie/Desktop/EFPro2/Calculation Results/MetanetX Mapping/chem_xref_2_alt.tsv.txt', delimiter='\t')
df_Kegg = chem_xref_MNX_2.loc[123406:151834,'XRef':'MNX_ID']
pivoted_Kegg = df_Kegg.pivot_table(index='XRef',columns='XRef_ID',values='MNX_ID',aggfunc = lambda x: x)
chem_xref_MNX_Kegg_dict = pivoted_Kegg.to_dict('index')
chem_xref_MNX_Kegg_dict = chem_xref_MNX_Kegg_dict['kegg']
###Output
_____no_output_____
###Markdown
Check how many Metabolites can be found in the MNX database and how many cannot be found at all.
###Code
print 'This is the total amount of metabolites in the model: %i' % (len(cameo_model.metabolites))
print 'We focus on mapping those %i metabolites in the model that have an MNX ID' % (len([met for met in cameo_model.metabolites if met.id.startswith('MNXM')]))
###Output
This is the total amount of metabolites in the model: 935
We focus on mapping those 270 metabolites in the model that have an MNX ID
###Markdown
How many of those MNX metabolites can we actually find in ver 2.0 of the MNX Dump?
###Code
not_in_chem_prop2 = []
in_chem_prop2 = []
for met in cameo_model.metabolites:
met_clean = ''.join(re.findall('(MNXM\d*)_',met.id))
if not met_clean == '':
if not met_clean in chem_prop_MNX_2_dict.keys():
not_in_chem_prop2.append(met)
else:
in_chem_prop2.append(met)
print '%i metabolites with MNX ID cannot be found in the most recent dump (ver 2.0) of the MNX database' % (len(not_in_chem_prop2))
print 'So lets check how many of these metabolites have a Kegg ID:'
print (len([met for met in cameo_model.metabolites if 'KEGG' in met.notes and met.notes['KEGG'] != '' and met.id.startswith('MNXM')]))
# Quick check-up to compare conditions before and after:
print len([met for met in cameo_model.metabolites if met.formula == ''])
print len([met for met in cameo_model.metabolites if met.formula == '' and met.id.startswith('MNXM')])
print len([met for met in cameo_model.metabolites if not met.charge])
print len([met for met in cameo_model.metabolites if not met.charge and met.id.startswith('MNXM')])
###Output
80
29
440
192
###Markdown
Match the model metabolites with the ver 2.0 of MNX via the KEGG IDs.
###Code
# No KEGG ID or the KEGG IDs don't match.
no_match_via_kegg = []
# Matched
jackpot = []
for met in not_in_chem_prop2:
if 'KEGG' in met.notes and met.notes['KEGG'] != '':
if met.notes['KEGG'][0] in chem_xref_MNX_Kegg_dict:
updated_mnx_id = chem_xref_MNX_Kegg_dict[met.notes['KEGG'][0]]
temp_id = met.id.split('_')
met.id = updated_mnx_id +'_'+ temp_id[1]
cameo_model.repair()
met.notes['MXNREF'] = [updated_mnx_id ]
jackpot.append(met)
else:
no_match_via_kegg.append(met)
else:
no_match_via_kegg.append(met)
print (len(jackpot), len(no_match_via_kegg))
###Output
(46, 6)
###Markdown
Add the metabolites that could be updated automatically to the list of metabolites that were matched to MNX ver 2.0 before
###Code
for met in jackpot:
in_chem_prop2.append(met)
# print (in_chem_prop2,len(in_chem_prop2))
no_match_via_kegg
# MNXM90227 changed to newer MNX ID: MNXM35. The Kegg ID matched to the one in the model
met = no_match_via_kegg[0]
met.charge = int(0)
met.notes['MXNREF'] = ['MNXM35']
met.id = 'MNXM35_c'
cameo_model.repair()
in_chem_prop2.append(met)
# MNXM54123 to the newer MNX ID: MNXM54124
# Changed on the basis of a finding KEGG Identifier C05796
met = no_match_via_kegg[1]
met.formula = 'C14H26O11'
met.charge = int(0)
met.notes['INCHI'] = ['InChI=1S/C14H26O11/c1-21-11-5(3-15)24-14(10(20)7(11)17)25-12-6(4-16)23-13(22-2)9(19)8(12)18/h5-20H,3-4H2,1-2H3/t5-,6-,7-,8-,9-,10-,11+,12+,13-,14+/m1/s1']
met.notes['SMILES'] = ['CO[C@@H]1O[C@H](CO)[C@H](O[C@@H]2O[C@H](CO)[C@H](OC)[C@H](O)[C@H]2O)[C@H](O)[C@H]1O']
met.notes['MXNREF'] = ['MNXM54124']
met.id = 'MNXM54124_c'
cameo_model.repair()
in_chem_prop2.append(met)
# MNXM3129 to the newer MNX ID: MNXM114594
# Changed on the basis of a finding KEGG Identifiers C04237 and C14463
met = no_match_via_kegg[2]
met.charge = int(-1)
met.notes['MXNREF'] = ['MNXM114594']
met.id = 'MNXM114594_c'
cameo_model.repair()
in_chem_prop2.append(met)
# MNXM1283 to the newer MNX ID: MNXM114238
# Changed on the basis of a finding KEGG Identifiers C04237 and C14463
met = no_match_via_kegg[3]
met.charge = int(0)
met.notes['MXNREF'] = ['MNXM114238']
met.id = 'MNXM114238_c'
cameo_model.repair()
in_chem_prop2.append(met)
# Cellulose, Left unchanged. A more accurate representation would be to measure the total content of cellulose
# per cell and then incorporate that into the biomass function, including a energy balances for
# degration/ construction terms. => Check how its done in other models
print no_match_via_kegg[4]
print no_match_via_kegg[4].notes
# MNXM89970 changed to newer MNX ID: MNXM1824. The Kegg ID matched to the one in the model but
# formula, INCHI, SMILES and other XREF IDs were changed. This Dolichyl phosphate is a more concrete example,
# and not a generic molecule of undetermined length.
met = no_match_via_kegg[5]
met.charge = int(-2)
met.formula = 'C80H131O4P'
met.notes['INCHI'] = ['InChI=1S/C80H133O4P/c1-65(2)33-18-34-66(3)35-19-36-67(4)37-20-38-68(5)39-21-40-69(6)41-22-42-70(7)43-23-44-71(8)45-24-46-72(9)47-25-48-73(10)49-26-50-74(11)51-27-52-75(12)53-28-54-76(13)55-29-56-77(14)57-30-58-78(15)59-31-60-79(16)61-32-62-80(17)63-64-84-85(81,82)83/h33,35,37,39,41,43,45,47,49,51,53,55,57,59,61,80H,18-32,34,36,38,40,42,44,46,48,50,52,54,56,58,60,62-64H2,1-17H3,(H2,81,82,83)/p-2/b66-35+,67-37+,68-39-,69-41-,70-43-,71-45-,72-47-,73-49-,74-51-,75-53-,76-55-,77-57-,78-59-,79-61-']
met.notes['SMILES'] = ['CC(CCOP([O-])([O-])=O)CC\C=C(\C)CC\C=C(\C)CC\C=C(\C)CC\C=C(\C)CC\C=C(\C)CC\C=C(\C)CC\C=C(\C)CC\C=C(\C)CC\C=C(\C)CC\C=C(\C)CC\C=C(\C)CC\C=C(\C)CC\C=C(/C)CC\C=C(/C)CCC=C(C)C']
met.notes['CHEBI'] = ['23875']
met.notes['METACYC'] = ['CPD-17833','DOLICHOLP']
met.notes['BIGG'] = ['dolp']
met.notes['SEED'] = ['cpd11619']
met.notes['BIOPATH'] = ['Dolichol-phosphate']
met.notes['LIPIDMAPS'] = ['LMPR03080015']
met.notes['HMDB'] = ['HMDB06353']
met.notes['REACTOME'] = ['162872','449299','449311']
met.notes['MXNREF'] = ['MNXM1824']
met.id = 'dolp_c'
cameo_model.repair()
###Output
_____no_output_____
###Markdown
For all the metabolites that could be found in the more recent database (ver. 2), get the Formula, Mass, ChargeIf one of those fields is empty in the model, fill it!
###Code
chem_prop_MNX_2_dict['MNXM105']
chem_xref_MNX_2_dict['MNXM105']
chem_xref_MNX_2_dict['MNXM105']['bigg'][0]
in_chem_prop2
form_update = 0
charge_update = 0
inchi_update = 0
smiles_update = 0
mnx2bigg_update = 0
for met in in_chem_prop2:
met_clean = ''.join(re.findall('(MNXM\d*)_',met.id))
if not met.charge and chem_prop_MNX_2_dict[met_clean]['Charge'] != 0:
if math.isnan(float(chem_prop_MNX_2_dict[met_clean]['Charge'])):
met.charge = int(0)
met.notes['Charge'] = int(0)
else:
met.charge = int(chem_prop_MNX_2_dict[met_clean]['Charge'])
met.notes['Charge'] = int(chem_prop_MNX_2_dict[met_clean]['Charge'])
charge_update += 1
if (met.formula == '' or re.findall('\(',met.formula)) and chem_prop_MNX_2_dict[met_clean]['Formula'] != '':
met.formula = str(chem_prop_MNX_2_dict[met_clean]['Formula'])
form_update += 1
if 'INCHI' in met.notes and met.notes['INCHI'] == '' and chem_prop_MNX_2_dict[met_clean]['InChi'] != '':
met.notes['INCHI'] = chem_prop_MNX_2_dict[met_clean]['InChi']
inchi_update += 1
if 'SMILES' in met.notes and met.notes['SMILES'] == '' and chem_prop_MNX_2_dict[met_clean]['SMILES'] != '':
met.notes['SMILES'] = chem_prop_MNX_2_dict[met_clean]['SMILES']
smiles_update += 1
if 'bigg:' in chem_prop_MNX_2_dict[met_clean]['Source']:
bigg_id = chem_prop_MNX_2_dict[met_clean]['Source'].split(':')[1]
temp_id = met.id.split('_')
met.id = bigg_id +'_'+ temp_id[1]
cameo_model.repair()
mnx2bigg_update += 1
print charge_update
print form_update
print inchi_update
print smiles_update
print mnx2bigg_update
# Quick check-up to compare conditions before and after:
print len([met for met in cameo_model.metabolites if met.formula == ''])
print len([met for met in cameo_model.metabolites if met.formula == '' and met.id.startswith('MNXM')])
print len([met for met in cameo_model.metabolites if not met.charge])
print len([met for met in cameo_model.metabolites if not met.charge and met.id.startswith('MNXM')])
###Output
51
0
424
176
###Markdown
How many MNXM IDs can be mapped directly to BIGG IDs.
###Code
MNX2Bigg_remapped_2 = 0
other_XRef_2 = []
x = float('NaN')
for met in in_chem_prop2:
met_clean = ''.join(re.findall('(MNXM\d*)_',met.id))
if met_clean in chem_xref_MNX_2_dict.keys() and type(chem_xref_MNX_2_dict[met_clean]['bigg']) != type(x) and chem_xref_MNX_2_dict[met_clean]['bigg'] != []:
bigg_id = chem_xref_MNX_2_dict[met_clean]['bigg'][0]
temp_id = met.id.split('_')
print bigg_id
met.id = str(bigg_id) +'_'+ temp_id[1]
cameo_model.repair()
MNX2Bigg_remapped_2 += 1
else:
other_XRef_2.append(met)
print '%i mets were successfully remapped from MNX to BIGG based on ver.2 MNX Chem XRef' % (MNX2Bigg_remapped_2)
print '%i metabolites with MNX ID cannot be found or can only be mapped to a different ID in ver. 2 ' % (len(other_XRef_2))
print 'There are a total of %i metabolites with MNX ID in the model' % (len([met for met in cameo_model.metabolites if met.id.startswith("MNX")]))
# Are these remaining Metabolites the same ones that cannot be found in the dumps?
ambiguous_matches = set([met for met in cameo_model.metabolites if met.id.startswith("MNX")]) - set(other_XRef_2)
# Check these out manually!!
for met in ambiguous_matches:
met_clean = ''.join(re.findall('(MNXM\d*)_',met.id))
print met_clean
other_XRef_2
###Output
_____no_output_____
###Markdown
Reactions REACTIONS: Check the notes section of each reaction for BIGG IDs first, replace all MNX IDs with those, then scavenge the MNX dumps for matches and get more BIGG IDs that way. Finally check reaction balance/ reversibility by going off of the BIGG Database itself!
###Code
metanetx_reactions = [rxn for rxn in cameo_model.reactions if rxn.id.startswith('MNXR')]
print len(metanetx_reactions)
print len(cameo_model.reactions)
rxn_mnx2bigg = []
rxn_nobiggid = []
for rxn in [rxn for rxn in cameo_model.reactions if rxn.id.startswith('MNXR')]:
if 'BIGG' in rxn.notes:
reaction_id = rxn.notes['BIGG'][0].split(', ')
tag_split = rxn.id.split('_')
rxn.id = str(reaction_id[0]) +'_'+ tag_split[1]
rxn_mnx2bigg.append(reaction_id)
cameo_model.repair()
else:
rxn_nobiggid.append(rxn)
print len(rxn_mnx2bigg)
print len(rxn_nobiggid)
metanetx_reactions = [rxn for rxn in cameo_model.reactions if rxn.id.startswith('MNXR')]
print len(metanetx_reactions)
print len(cameo_model.reactions)
reac_xref_MNX_1 = read_csv('/Users/clie/Desktop/EFPro2/Calculation Results/MetanetX Mapping/reac_xref_1_alt.tsv.txt', delimiter='\t')
df_1 = reac_xref_MNX_1.loc[:,'XRef':'MNX_ID']
groups = df_1.groupby(['MNX_ID','XRef'])
df_1 = groups.apply(lambda x:list(x['XRef_ID']))
df_1 =df_1.unstack('XRef')
reac_xref_MNX_1_dict = df_1.to_dict('index')
reac_xref_MNX_2 = read_csv('/Users/clie/Desktop/EFPro2/Calculation Results/MetanetX Mapping/reac_xref_2_alt.tsv.txt', delimiter='\t')
df_2 = reac_xref_MNX_2.loc[:,'XRef':'MNX_ID']
groups = df_2.groupby(['MNX_ID','XRef'])
df_2 = groups.apply(lambda x:list(x['XRef_ID']))
df_2 =df_2.unstack('XRef')
reac_xref_MNX_2_dict = df_2.to_dict('index')
reac_property_MNX = read_csv('/Users/clie/Desktop/EFPro2/Calculation Results/MetanetX Mapping/reac_prop_noheader.tsv.txt', delimiter='\t')
reac_property_MNX.set_index('MNX_ID',inplace = True)
df_3 = reac_property_MNX.fillna('MISSING')
reac_property_MNX_dict = df_3.to_dict('index')
rxn_ID_not_in_MetaNetX_2 = [rxn for rxn in rxn_nobiggid if rxn.id.startswith('MNXR') and ''.join(re.findall('(MNXR\d*)_',rxn.id)) not in reac_xref_MNX_2_dict.keys()]
print len(rxn_ID_not_in_MetaNetX_2)
# Get a mapping from KEGG to the new MNXR IDs, for those reactions that cannot be found in the MNX Database,
# but that have a KEGG ID in their annotation.
df_2 = reac_xref_MNX_2.loc[13004:22928,'XRef_ID':'MNX_ID']
df_2 = df_2.set_index(['XRef_ID'])
kegg_2_mnx_dict_2 = df_2.to_dict('index')
###Output
_____no_output_____
###Markdown
Try to find a KEGG ID in the notes section of those reactions that do not have a BIGG ID, but whose MNX IDs also don't exist in either ver 1.0 or 2.0 of the MNX Database.
###Code
for rxn in rxn_nobiggid:
print rxn.notes
duplicate_entries_check_up = []
kegg_not_in_ver2_of_mnx = []
no_kegg_no_bigg_no_realMNX = []
kegg_present = 0
for rxn in rxn_nobiggid:
if rxn.notes['KEGG'] != []:
kegg_present += 1
if ',' not in rxn.notes['KEGG'][0]:
kegg_id_in_model = rxn.notes['KEGG'][0]
if kegg_id_in_model in kegg_2_mnx_dict_2:
ver2_MNX_ID = kegg_2_mnx_dict_2[kegg_id_in_model]
just_MNX_ID = ver2_MNX_ID['MNX_ID']
rxn.notes['MXNREF'] = [just_MNX_ID]
temp_id = rxn.id.split('_')
rxn.id = str(just_MNX_ID) +'_'+ temp_id[1]
cameo_model.repair()
else:
kegg_not_in_ver2_of_mnx.append(rxn)
else:
duplicate_entries_check_up.append(rxn)
else:
no_kegg_no_bigg_no_realMNX.append(rxn)
print len(duplicate_entries_check_up),kegg_present, len(kegg_not_in_ver2_of_mnx) ,len(no_kegg_no_bigg_no_realMNX)
###Output
19 390 3 0
###Markdown
Manual check-up of the duplicate entries:
###Code
# Fructose-Bisphospate Aldolase has two KEGG IDs: One operating on both isomers of fdp and one on beta_fructose only.
# The former was chosen since it is annotated to be part of the methane metabolism
# Hence MNX ID MNXR2184 was swapped with the newer MNXR13125
rxn = duplicate_entries_check_up[0]
rxn.notes['KEGG'] = ['R01068']
rxn.notes['MXNREF'] = ['MNXR13125']
rxn.id = 'MNXR13125_c'
cameo_model.repair()
# Transketolase has two KEGG IDs: One operating on both isomers of f6p and one on beta_fructose only.
# The former was chosen since it is annotated to be part of the methane metabolism
# Hence MNX ID MNXR35646 was swapped with the newer MNXR84331
rxn = duplicate_entries_check_up[1]
rxn.notes['KEGG'] = ['R01067']
rxn.notes['MXNREF'] = ['MNXR84331']
rxn.id = 'MNXR84331_c'
cameo_model.repair()
# Transaldolase has two KEGG IDs: One operating on beta_fructose only and the other on both isomers of fructose f6p.
# The former was chosen since it is annotated to be part of the methane metabolism
# Hence MNX ID MNXR35645 was swapped with the newer MNXR84303
rxn = duplicate_entries_check_up[2]
rxn.notes['KEGG'] = ['R08575']
rxn.notes['MXNREF'] = ['MNXR84303']
rxn.id = 'MNXR84303_c'
cameo_model.repair()
# D-arabino-hex-3-ulose-6-phosphate isomerase has two KEGG IDs: One operating on beta_fructose only and the other on both isomers of fructose f6p.
# The former was chosen since it is annotated to be part of the methane metabolism
# Hence MNX ID MNXR36673 was swapped with the newer MNXR85335
rxn = duplicate_entries_check_up[3]
rxn.notes['KEGG'] = ['R05339']
rxn.notes['MXNREF'] = ['MNXR85335']
rxn.id = 'MNXR85335_c'
cameo_model.repair()
# MNXID MNXR59222 was swapped with the newer MNXR73725
# Both KEGG IDs in the model are united in the new MNX ID
rxn = duplicate_entries_check_up[4]
rxn.notes['MXNREF'] = ['MNXR73725']
rxn.id = 'MNXR73725_c'
cameo_model.repair()
# Already the most recent MNX ID. Left unchanged.
duplicate_entries_check_up[5]
# MNXID MNXR57244 was swapped with the newer MNXR71471
# Both KEGG IDs in the model are united in the new MNX ID
rxn = duplicate_entries_check_up[6]
rxn.notes['MXNREF'] = ['MNXR71471']
rxn.id = 'MNXR71471_c'
cameo_model.repair()
# MNXID MNXR6714 was swapped with the newer MNXR74397
# I chose KEGG ID 'R05071' because there was a BIGG ID available for it. The other KEGG ID 'R03052' differed only
# in the EC Number and in the common name of the metabolites.
rxn = duplicate_entries_check_up[7]
rxn.notes['KEGG'] = ['R05071']
rxn.notes['MXNREF'] = ['MNXR74397']
rxn.id = 'MNXR74397_c'
cameo_model.repair()
# MNXID MNXR35780 was swapped with the newer MNXR84997
# Both KEGG IDs in the model are united in the new MNX ID
rxn = duplicate_entries_check_up[8]
rxn.id = 'MNXR84997_c'
cameo_model.repair()
# Already the most recent MNX ID. Left unchanged.
duplicate_entries_check_up[9]
# Already the most recent MNX ID. Left unchanged.
duplicate_entries_check_up[10]
# Already the most recent MNX ID. Left unchanged.
duplicate_entries_check_up[11]
# Already the most recent MNX ID. Left unchanged.
duplicate_entries_check_up[12]
# Already the most recent MNX ID. Left unchanged.
duplicate_entries_check_up[13]
# Already the most recent MNX ID. Left unchanged.
duplicate_entries_check_up[14]
# Already the most recent MNX ID. Left unchanged.
duplicate_entries_check_up[15]
# MNXID MNXR56370 was swapped with the newer MNXR70454
# I chose KEGG ID 'R04672' because there was a BIGG ID available for it. The other KEGG ID 'R03050' differed
# only in the direction of the reaction. EC number and metabolites are identical
rxn = duplicate_entries_check_up[16]
rxn.notes['KEGG'] = ['R04672']
rxn.notes['MXNREF'] = ['MNXR70454']
rxn.id = 'MNXR70454_c'
cameo_model.repair()
# Already the most recent MNX ID. Left unchanged.
duplicate_entries_check_up[17]
# MNXID MNXR39690 was swapped with the newer MNXR83628
# I chose KEGG ID 'R00764' because there was a BIGG ID available for it. The other KEGG ID 'R02073' differed
# only in the type of Fructose. Again the beta-fructose and the metabolite that allows either
# isoform.
rxn = duplicate_entries_check_up[18]
rxn.notes['KEGG'] = ['R00764']
rxn.notes['MXNREF'] = ['MNXR83628']
rxn.id = 'MNXR83628_c'
cameo_model.repair()
rxn_ID_not_in_MetaNetX_2 = [rxn for rxn in rxn_nobiggid if rxn.id.startswith('MNXR') and ''.join(re.findall('(MNXR\d*)_',rxn.id)) not in reac_xref_MNX_2_dict.keys()]
print len(rxn_ID_not_in_MetaNetX_2)
# MNXID MNXR14767 was swapped with the newer MNXR68614
# Everything was transferred over from MNX since not even the KEGG ID seemed to exist.
# This reaction was found by searching for the reaction string on MetanetX
rxn = rxn_ID_not_in_MetaNetX_2[0]
rxn.notes['MXNREF'] = ['MNXR68614']
rxn.notes['KEGG'] = ['R01286']
rxn.notes['RHEA'] = ['13965','13966','13967','13968']
rxn.notes['METACYC'] = ['CYSTATHIONINE-BETA-LYASE-RXN']
rxn.notes['BIOPATH'] = ['RXN00195']
rxn.notes['UPA'] = ['UCR01286','UER00078']
rxn.id = 'MNXR68614_c'
cameo_model.repair()
rxn_ID_not_in_MetaNetX_2 = [rxn for rxn in rxn_nobiggid if rxn.id.startswith('MNXR') and ''.join(re.findall('(MNXR\d*)_',rxn.id)) not in reac_xref_MNX_2_dict.keys()]
print len(rxn_ID_not_in_MetaNetX_2)
rxn_MNX2Bigg_remapped_2 = 0
rxn_other_XRef_2 = []
rxn_MNX2Bigg_remapped_1 = 0
rxn_other_XRef_1 = []
x = float('NaN')
for rxn in rxn_nobiggid:
rxn_clean = ''.join(re.findall('(MNXR\d*)_',rxn.id))
if rxn_clean in reac_xref_MNX_2_dict.keys() and type(reac_xref_MNX_2_dict[rxn_clean]['bigg']) != type(x) and reac_xref_MNX_2_dict[rxn_clean]['bigg'] != []:
bigg_id = reac_xref_MNX_2_dict[rxn_clean]['bigg'][0]
print bigg_id
rxn.notes['BIGG'] = [bigg_id]
temp_id = rxn.id.split('_')
rxn.id = str(bigg_id) +'_'+ temp_id[1]
cameo_model.repair()
rxn_MNX2Bigg_remapped_2 += 1
else:
rxn_other_XRef_2.append(rxn)
print '%i rxn were successfully remapped from MNX to BIGG based on ver.2 MNX Reac XRef' % (rxn_MNX2Bigg_remapped_2)
print '%i reactions with MNX ID cannot be found or can only be mapped to a different ID in ver. 2 ' % (len(rxn_other_XRef_2))
###Output
FBA
PDHam1hi
GAPD
TPI
PGIA
PGCM
G6PI_1
GLUKA
EDA
TKT2
TALA
3HAD40_1
3OAR40_1
BTNC
ACCOAC_1
DSMSTOLR
DDSMSTOLR
CHLSTR
ZYMSTR
DMTPHT
GTPOPm
AGPOP
DAPOP
AIRC2
CBPS
DHORDfum
SGAT
SERH
GLYTA
ARD_1
ACDO_co
MHPGLUT
DMT
2OH3K5MPPISO
CYSS_trdrd
CITCIb
KARI_23dhmp
KARI
KARI_3hmoa
KARI_23dhmb
ACODA
ORNTAC
ORNTA
CSPMDDC
G3PL
AROH
APCS
SAGH
UGE
UAGDP
G1PACT
AHEXASE3
ACCOAC
RBCh
APLh
FGFTh
DHFR2i
DHFOR
THFOR1
THFOR2
NAD_H2
PRUK
NMNHYD
APNPT
APCPT
PMEACPE
AOXSr2
OGMEACPD
EGMEACPR
OPMEACPD
EPMEACPR
CPMPS
6CTDS
CDGR
CODSCL5BMT
DXPS
DMPPS_syn
IDS1
PSPPS
SS
SQLC
SQLC2
HCO3E
GLNTRAT
ASNTRAT
3OACOAR
COA1819ZD9DS
ASPO2y
GAPDH_nadp_hi
PRDX
ALCD1
DHORD_NAD
PC
ASPTA6
MOD_3mop
PFK_ppi
NITR
97 rxn were successfully remapped from MNX to BIGG based on ver.2 MNX Reac XRef
293 reactions with MNX ID cannot be found or can only be mapped to a different ID in ver. 2
###Markdown
Final Metabolite formula cleanup. All formulas that contain brackets are set to empty strings. They will have to be checked up later on!
###Code
poly_forumla = []
for met in cameo_model.metabolites:
if re.findall('\(',met.formula):
if 'MXNREF' in met.notes and met.notes['MXNREF'] != ['']:
if met.notes['MXNREF'][0] in chem_prop_MNX_2_dict:
print chem_prop_MNX_2_dict[met.notes['MXNREF'][0]]['Formula']
poly_forumla.append(met)
met.formula = str()
else:
print met
poly_forumla
###Output
_____no_output_____
###Markdown
In/ Out Reactions that have Metabolite names as their IDs are updated
###Code
RXN_MNX2Bigg_remapped_2 = 0
other_XRef_2 = []
x = float('NaN')
for rxn in cameo_model.reactions:
rxn_clean = ''.join(re.findall('(MNXM\d*)_',rxn.id))
if rxn_clean in chem_xref_MNX_2_dict.keys() and type(chem_xref_MNX_2_dict[rxn_clean]['bigg']) != type(x) and chem_xref_MNX_2_dict[rxn_clean]['bigg'] != []:
bigg_id = chem_xref_MNX_2_dict[rxn_clean]['bigg'][0]
temp_id = rxn.id.split('_')
print bigg_id,rxn.id
rxn.id = str(bigg_id) +'_'+ temp_id[1]
cameo_model.repair()
RXN_MNX2Bigg_remapped_2 += 1
else:
other_XRef_2.append(met)
print '%i mets were successfully remapped from MNX to BIGG based on ver.2 MNX Chem XRef' % (RXN_MNX2Bigg_remapped_2)
print '%i metabolites with MNX ID cannot be found or can only be mapped to a different ID in ver. 2 ' % (len(other_XRef_2))
cameo_model.reactions.f1p_out.reactants[0].id.split('_')
in_out_rxns = [rxn for rxn in cameo_model.reactions if rxn.id.endswith('out') or rxn.id.endswith('in')]
for rxn in in_out_rxns:
if rxn.id.startswith('MNX'):
id_part = rxn.reactants[0].id.split('_')
tag_part = rxn.id.split('_')
rxn.id = id_part[0]+'_' +tag_part[1]
print rxn.id
###Output
g3p_out
2ahethmpp_out
MNXM6145_out
MNXM90032_out
MNXM11251_out
MNXM8480_out
MNXM24_out
MNXM35_out
MNXM3480_out
MNXM5590_out
MNXM1659_out
MNXM54124_out
MNXM2573_out
MNXM6439_out
MNXM25932_out
3hbutACP_out
MNXM277_out
MNXM832_out
MNXM35647_out
MNXM3231_out
MNXM5534_out
MNXM57949_out
MNXM3359_out
mergtrol_out
MNXM2144_out
MNXM4307_out
MNXM1036_out
MNXM6075_out
MNXM6758_out
MNXM5489_out
MNXM5000_out
MNXM90278_out
MNXM9890_out
MNXM5555_out
MNXM77745_out
dmtphllqne_out
MNXM97071_out
MNXM715_out
MNXM9892_out
MNXM9891_out
MNXM39835_out
MNXM221_out
MNXM201_out
MNXM18_out
3hmop_out
MNXM1421_out
MNXM30985_out
MNXM265_out
MNXM774_out
S_out
MNXM126482_out
MNXM72_out
MNXM93768_out
MNXM101_out
MNXM97048_out
MNXM1762_out
MNXM6202_out
MNXM2217_out
MNXM3314_out
MNXM4010_out
MNXM2365_out
MNXM6572_out
MNXM2643_out
MNXM6665_out
MNXM3436_out
MNXM16361_out
MNXM10825_out
MNXM10142_out
MNXM10203_out
MNXM14895_out
MNXM38125_out
MNXM81829_out
MNXM2429_out
MNXM5818_out
MNXM19347_out
MNXM90693_out
MNXM11629_out
MNXM1014_out
MNXM4035_out
MNXM2066_out
MNXM8633_out
MNXM4482_out
MNXM2041_out
MNXM12137_out
MNXM3012_out
MNXM768_out
MNXM2165_out
MNXM114238_out
MNXM4201_out
MNXM7734_out
MNXM1948_out
MNXM3350_out
MNXM381_out
MNXM2091_out
MNXM56489_out
MNXM6428_out
MNXM90460_out
MNXM7243_out
MNXM60163_out
MNXM1003_out
MNXM2656_out
MNXM2657_out
MNXM4551_out
MNXM89653_out
MNXM109_out
MNXM226_out
MNXM110_out
ptd2meeta_out
MNXM32126_out
MNXM2059_out
MNXM38414_out
MNXM484_out
MNXM413_out
MNXM4377_out
MNXM2809_out
MNXM2294_out
MNXM6875_out
MNXM5360_out
MNXM1952_out
MNXM2330_out
MNXM3206_out
MNXM977_out
MNXM5312_out
MNXM6087_out
MNXM6216_out
MNXM5676_out
MNXM1816_out
MNXM2318_out
MNXM1198_out
MNXM6043_out
MNXM6494_out
MNXM4972_out
MNXM4910_out
MNXM3867_out
MNXM722_out
MNXM5853_out
MNXM741_out
MNXM16389_out
MNXM12638_out
MNXM43631_out
MNXM3919_out
MNXM1611_out
MNXM2914_out
MNXM6678_out
MNXM2475_out
MNXM3440_out
MNXM1803_out
MNXM5283_out
MNXM5993_out
ficytC_out
MNXM667_out
MNXM809_out
MNXM1436_out
MNXM1087_out
MNXM1050_out
MNXM5649_out
MNXM73306_out
MNXM3891_out
MNXM1979_out
MNXM1213_out
MNXM96175_out
MNXM861_out
MNXM4111_out
pimACP_out
pmeACP_out
MNXM97572_out
MNXM10051_out
egmeACP_out
gmeACP_out
MNXM10052_out
hpmeACP_out
epmeACP_out
MNXM19093_out
MNXM96070_out
MNXM91491_out
MNXM4090_out
MNXM13005_out
MNXM4269_out
MNXM53766_out
MNXM1193_out
MNXM93263_out
codscl5b_out
MNXM21342_out
MNXM5285_out
MNXM7549_out
MNXM4463_out
MNXM6386_out
MNXM2142_out
MNXM3707_out
MNXM4076_out
MNXM7231_out
MNXM95609_out
MNXM96150_out
MNXM2499_out
MNXM92688_out
dolp_out
MNXM1740_out
MNXM2120_out
MNXM3563_out
MNXM4297_out
MNXM1682_out
MNXM90307_out
2mhob_out
MNXM5588_out
2mbcoa_out
MNXM12690_out
MNXM1293_out
MNXM5412_out
MNXM5511_out
MNXM3034_out
MNXM6152_out
MNXM6177_out
MNXM1651_out
MNXM1731_out
MNXM4764_out
MNXM2354_out
MNXM2709_out
MNXM114652_out
MNXM1289_out
###Markdown
Add in EC Numbers from the latest MNX reaction properties dump
###Code
for rxn in cameo_model.reactions:
if rxn.id.endswith('_c'):
mnx_id = rxn.notes['MXNREF'][0]
if (mnx_id in reac_property_MNX_dict.keys()) and (reac_property_MNX_dict[mnx_id]['EC'] != 'MISSING'):
rxn.notes.update({'EC Number':[reac_property_MNX_dict[mnx_id]['EC']]})
print rxn.id, rxn.notes['EC Number'][0]
###Output
FBA_c 4.1.2.13
ALCD2if_c 1.1.1.1;1.1.1.71
PDHam1hi_c 1.2.4.1;2.2.1.6;4.1.1.1
MNXR85044_c 1.2.4.1
MNXR73919_c 2.3.1.12
PYK_c 2.7.1.40
ENO_c 4.2.1.11
PGM_c 5.4.2.1;5.4.2.11;5.4.2.12
GAPD_c 1.2.1.12;1.2.1.59
TPI_c 5.3.1.1
PFK_c 2.7.1.11
PGIA_c 5.3.1.9
PGCM_c 5.4.2.2;5.4.2.5
PGI_c 5.3.1.9
MNXR5673_c 2.7.1.1;2.7.1.2
G6PI_1_c 5.1.3.15;5.3.1.9
GLUKA_c 2.7.1.1;2.7.1.2
MNXR85631_c 1.8.1.4
PGK_c 2.7.2.3
MNXR61040_c 1.1.2.7
ACS_c 6.2.1.1
MNXR26374_c 1.3.99.-
MNXR70375_c 1.2.4.2;2.5.1.64
MNXR85055_c 1.2.4.2
MNXR73920_c 2.3.1.61
SUCOAS_c 6.2.1.5
ICDHxm_c 1.1.1.286;1.1.1.41
FUM_c 4.2.1.2
ACONTb_c 4.2.1.3
ACONTa_c 4.2.1.3
CS_c 2.3.3.1;2.3.3.16;2.3.3.3
MDH_c 1.1.1.299;1.1.1.37
EDA_c 4.1.2.14;4.1.2.55
MNXR79510_c 2.2.1.1
RPE_c 5.1.3.1
TKT2_c 2.2.1.1
GND_c 1.1.1.351;1.1.1.44
PGL_c 3.1.1.31
G6PDH2_c 1.1.1.363;1.1.1.49
EDD_c 4.2.1.12
TALA_c 2.2.1.2
MNXR79509_c 4.1.2.9
MNXR5933_c 4.1.2.-;4.1.2.43
MNXR85335_c 5.3.1.27
MAN6PI_c 5.3.1.8
PMANM_c 5.4.2.8
GFUCS_c 1.1.1.271
GMAND_c 4.2.1.47
MAN1PT_c 2.7.7.13
FBA2_c 4.1.2.13
PFK_2_c 2.7.1.11;2.7.1.144;2.7.1.56
MNXR74047_c 2.7.1.11
MNXR74048_c 2.7.1.11
MNXR74049_c 2.7.1.11
MNXR73725_c 3.2.1.23
MNXR85653_c 1.3.1.-;1.3.1.9;2.3.1.-
MNXR148_c 2.3.1.-;4.2.1.-
MNXR85652_c 2.3.1.-;2.3.1.179;2.3.1.199;2.3.1.41;2.3.1.86
MNXR68187_c 1.1.1.100;2.3.1.-
ACOATA_c 2.3.1.-;2.3.1.38;2.3.1.85;2.3.1.86
MNXR74372_c 1.3.1.9;2.3.1.-
EAR40x_c 1.3.1.9;2.3.1.-
3HAD40_1_c 2.3.1.-;2.3.1.85;2.3.1.86;4.2.1.59
3OAR40_1_c 1.1.1.100;2.3.1.-;2.3.1.85;2.3.1.86
MCOATA_c 2.3.1.-;2.3.1.39;2.3.1.85;2.3.1.86
BTNC_c 6.3.4.14
ACCOAC_1_c 6.4.1.2
MNXR5765_c 1.1.1.1;1.1.1.71;1.2.1
FACOAL160_c 6.2.1.3
MNXR75097_c 1.3.1.72
DSMSTOLR_c 1.3.1.72
DDSMSTOLR_c 1.3.1.72
CHLSTR_c 1.3.1.72
ZYMSTR_c 1.3.1.72
MNXR17346_c 1.3.1.72
MNXR85611_c 1.3.1.72
MNXR6796_c 1.14.13.70
MNXR15403_c 1.3.1.72
MNXR3255_c 1.14.13.70
MNXR18371_c 2.1.1.222
OHPHM_c 2.1.1.222
MNXR18370_c 1.14.13.-
MNXR15867_c 1.14.13;1.14.13.-
MNXR59754_c 4.1.1.98
OPHBDC_c 4.1.1.98
MNXR60921_c 2.1.1.64
MNXR85363_c 2.1.1.64
CHRPL_c 4.1.3.40
MNXR85295_c 2.5.1.39
HBZOPT_c 2.5.1.39
DMTPHT_c 2.1.1.163
MNXR59755_c 2.1.1.163
MNXR18374_c 1.14.13.-
MNXR36135_c 1.14.13.-
MNXR75548_c 2.1.1.201
URFGTT_c 2.1.1.163;2.1.1.201
MNXR60918_c 1.14.13.-
MNXR60025_c 1.14.13.-
PRAGS_c 6.3.4.13
GARFT_c 2.1.2.2
AICART_c 2.1.2.3
ADSL2_c 4.3.2.2
MNXR84768_c 2.7.7.8
MNXR84766_c 2.7.7.19;2.7.7.48;2.7.7.6
MNXR14582_c 2.7.7.7
NDPK8_c 2.7.4.6
ADK1_c 2.7.4.3
ADSL1_c 4.3.2.2
NTD7_c 3.1.3.5
ADNK1_c 2.7.1.20;2.7.1.74
NTD6_c 3.1.3.5;3.1.3.89
NTD11_c 3.1.3.5
IMPD_c 1.1.1.205
GMPS_c 6.3.5.2
GMPS2_c 6.3.4.1;6.3.5.2
NTD9_c 3.1.3.5
NTD10_c 3.1.3.5
MNXR84770_c 2.7.7.8
NTD8_c 3.1.3.5;3.1.3.89
MNXR84772_c 2.7.7.48;2.7.7.6
MNXR14583_c 2.7.7.7
AP4AH_c 3.6.1.41
SADT_c 2.7.7.4
ADSK_c 2.7.1.25
PRFGS_c 6.3.5.3
NTPP9_c 3.6.1.19;3.6.1.8
NTPP2_c 3.6.1.19;3.6.1.8
NTPP11_c 3.6.1.19;3.6.1.66
NTPP1_c 3.6.1.19
NTPP6_c 3.6.1.8
ATPM_c 3.6.1.15;3.6.1.3;3.6.1.5;3.6.1.8;3.6.3.1;3.6.3.10;3.6.3.11;3.6.3.12;3.6.3.14;3.6.3.15;3.6.3.16;3.6.3.17;3.6.3.18;3.6.3.19;3.6.3.2;3.6.3.20;3.6.3.21;3.6.3.22;3.6.3.23;3.6.3.24;3.6.3.25;3.6.3.26;3.6.3.27;3.6.3.28;3.6.3.29;3.6.3.3;3.6.3.30;3.6.3.31;3.6.3.32;3.6.3.33;3.6.3.34;3.6.3.35;3.6.3.36;3.6.3.37;3.6.3.38;3.6.3.39;3.6.3.4;3.6.3.40;3.6.3.41;3.6.3.42;3.6.3.43;3.6.3.44;3.6.3.46;3.6.3.47;3.6.3.48;3.6.3.49;3.6.3.5;3.6.3.50;3.6.3.51;3.6.3.52;3.6.3.53;3.6.3.54;3.6.3.6;3.6.3.7;3.6.3.8;3.6.3.9;3.6.4.1;3.6.4.10;3.6.4.11;3.6.4.12;3.6.4.13;3.6.4.2;3.6.4.3;3.6.4.4;3.6.4.5;3.6.4.6;3.6.4.7;3.6.4.8;3.6.4.9
GTPOPm_c 2.7.1.40
AGPOP_c 2.7.1.40
DAPOP_c 2.7.1.40
IMPC_c 2.1.2.3;3.5.4.10
AIRC2_c 6.3.4.18
CBPS_c 6.3.5.5
ASPCT_c 2.1.3.2
DHORTS_c 3.5.2.3
DHORDfum_c 1.3.98.1
MNXR84769_c 2.7.7.8
MNXR84774_c 2.7.7.48;2.7.7.52;2.7.7.6
DCTPD2_c 3.5.4.13
CTPS1_c 6.3.4.2
CTPS2_c 6.3.4.2
NTD2_c 3.1.3.5
MNXR84773_c 2.7.7.48;2.7.7.6
MNXR84771_c 2.7.7.8
NTD4_c 3.1.3.5;3.1.3.91
CSND_c 3.5.4.1
DCTPD_c 3.5.4.13;3.5.4.14
MNXR14584_c 2.7.7.7
NTD3_c 3.1.3.5;3.1.3.89
DUTPDP_c 3.6.1.19;3.6.1.23
DURIPP_c 2.4.2.1;2.4.2.2;2.4.2.3;2.4.2.4
TMDS_c 2.1.1.45
MNXR14585_c 2.7.7.7
NTD5_c 3.1.3.35;3.1.3.5;3.1.3.89
TMDPP_c 2.4.2.2;2.4.2.4
MNXR9867_c 3.5.4.1
OMPDC_c 4.1.1.23
NTPP8_c 3.6.1.19;3.6.1.8
NTPP4_c 3.6.1.19;3.6.1.65;3.6.1.8
ASNS1_c 6.3.5.4
ASPO1_c 1.4.3.16;1.4.3.2
ASPTA_c 2.6.1.1
ARGSS_c 6.3.4.5
ARGSL_c 4.3.2.1
AGT_c 2.6.1.44
ALAD_L_c 1.4.1.1
SSALx_c 1.2.1.16;1.2.1.24
SSALy_c 1.2.1.16;1.2.1.79
GLUSy_c 1.4.1.13
GLUSx_c 1.4.1.14
GLUDx_c 1.4.1.2;1.4.1.3
GLUDy_c 1.4.1.13;1.4.1.3;1.4.1.4
GLNS_c 6.3.1.2
GF6PTA_c 2.6.1.16
GLYCK_c 2.7.1.31
SGAT_c 2.6.1.45
SPTix_c 2.6.1.51
ASPK_c 2.7.2.4
PSERT_c 2.6.1.52
PSP_L_c 3.1.3.3
GHMT2_c 2.1.2.1
THRD_L_c 4.3.1.19
THRS_c 4.2.3.1
ASAD_c 1.2.1.11
GCCa_c 1.4.4.2
HSDx_c 1.1.1.3
HSDy_c 1.1.1.3
GCCb_c 2.1.2.10
GCCc_c 1.8.1.4
SERD_L_c 4.3.1.17;4.3.1.19
MNXR69809_c 2.7.8.8
SERH_c 4.2.1.20
GLYTA_c 2.6.1.4;2.6.1.44
SHSL2r_c 2.5.1.48;4.2.99.9
ARD_1_c 1.13.11.54
ACDO_co_c 1.13.11.53
MNXR3469_c 4.2.1.109
MTRI_c 5.3.1.23
MTAP_c 2.4.2.28
SPMS_c 2.5.1.16
ADMDC_c 4.1.1.50
METS_c 2.1.1.13;2.1.1.14
MHPGLUT_c 2.1.1.14
METAT_c 2.5.1.6
AHC_c 3.3.1.1
DMT_c 2.1.1.37
AHCYSNS_c 3.2.2.9
2OH3K5MPPISO_c 3.1.3.77;3.1.3.87
CYSS_c 2.5.1.47;2.5.1.65
SERAT_c 2.3.1.30
CYSS_trdrd_c 2.5.1.47;2.5.1.49
CYSS2_c 2.5.1.47
LCYSTAT_c 2.6.1.1
CYSLYSL_c 4.4.1.1;4.4.1.8
3SALATAi_c 2.6.1;2.6.1.1
CYSDS_c 4.4.1.1;4.4.1.28;4.4.1.8
MNXR70329_c 2.8.1.2
MNXR1357_c 2.6.1.1
HSST_c 2.3.1.46
VALTA_c 2.6.1.42;2.6.1.6
ILETA_c 2.6.1.42
LEUTA_c 2.6.1.42;2.6.1.6;2.6.1.67
CITCIb_c 4.2.1.35
MNXR14719_c 1.1.1;1.1.1.85
KARI_23dhmp_c 1.1.1.86
IPPMIb_c 4.2.1.33
IPMD_c 1.1.1.85
IPPMIa_c 4.2.1.33
IPPS_c 2.3.3.13
DHAD1_c 4.2.1.9
KARI_c 1.1.1.86;5.4.99.3
KARI_3hmoa_c 1.1.1.86;5.4.99.3
DHAD2_c 4.2.1.9
KARI_23dhmb_c 1.1.1.86
MNXR7636_c 4.2.1.35
ACLS_c 2.2.1.6
UGMDDS_c 6.3.2.10
MNXR61396_c 4.3.3.7
MNXR65723_c 1.17.1.8
DHDPRy_c 1.3.1.26
THDPS_c 2.3.1.117
SDPTA_c 2.6.1.17
SDPDS_c 3.5.1.18
DAPE_c 5.1.1.7
DAPDC_c 4.1.1.20
HCITS_c 2.3.3.14
UAAGDS_c 6.3.2.13
MNXR15177_c 2.6.1.21
MNXR84891_c 1.2.4.2
MNXR84961_c 2.3.1.61
MNXR84920_c 3.5.1.11
MNXR85427_c 3.5.2.6
ACGS_c 2.1.4.1;2.3.1.1
ACGK_c 2.7.2.8
AGPR_c 1.2.1.38
ACOTA_c 2.6.1.11
ACODA_c 3.5.1.14;3.5.1.16
ORNTAC_c 2.3.1.35
ORNTA_c 2.6.1.13
MNXR59113_c 3.4.11.5
MNXR74389_c 2.6.1.1;2.6.1.23
MNXR74390_c 2.6.1.21
MNXR56317_c 4.1.3.16
ARGDC_c 4.1.1.19
MNXR6619_c 3.5.1.53
GLU5K_c 2.7.2.11
G5SD_c 1.2.1.41
MNXR5481_c 3.5.4.1;3.5.4.21
CSPMDDC_c 4.1.1;4.1.1.96
MNXR61035_c 4.1.1.96
MNXR8886_c 3.5.1.16
SPRMS_c 2.5.1.16;2.5.1.22
HISDC_c 4.1.1.22;4.1.1.28
MNXR59227_c 1.1.1.23
MNXR58251_c 1.1.1.23
HSTPT_c 2.6.1.9
IGPDH_c 4.2.1.19
PRMICI_c 5.3.1.16
PRAMPC_c 3.5.4.19
PRATPP_c 3.6.1.31
IG3PS_c 2.4.2;2.4.2.-;4.1.3.-
34DHOXPEGOX_c 1.1.1.1
TYRTA_c 2.6.1.1;2.6.1.5;2.6.1.57;2.6.1.9
PACCOAL_c 6.2.1.30
PHETA1_c 2.6.1.1;2.6.1.5;2.6.1.57;2.6.1.9
MNXR14849_c 2.6.1.21
CINNDO_c 1.14.12.19
PPPNDO_c 1.14.12.19
MNXR16964_c 1.14.-.-
MNXR16969_c 1.14.-.-
MNXR18597_c 1.14.-.-
MNXR18599_c 1.14.-.-
INDPYRD_c 4.1.1.43;4.1.1.74
2OXOADOXm_c 1.2.4.2;1.8.1.4;2.3.1.61
DDPA_c 2.5.1.54
CHORS_c 4.2.3.5
G3PL_c 4.1.2.8;4.2.1.20
TRPS2_c 4.2.1.122;4.2.1.20
IGPS_c 4.1.1.48
PRAI_c 5.3.1.24
ANS2_c 4.1.3.27
ANS_c 4.1.3.27
CHORM_c 5.4.99.5
PPNDH_c 1.3.1.12;4.2.1.51;4.2.1.91
AROH_c 4.2.1.51;4.2.1.91
PPND_c 1.3.1.12
PSCVT_c 2.5.1.19
SHKK_c 2.7.1.71
SHK3D_c 1.1.1.25;1.1.1.282
DHQD_c 4.2.1.10
DHQS_c 4.2.3.4
PANTS_c 6.3.2.1
ASP1DC_c 4.1.1.11;4.1.1.15
MNXR73792_c 2.3.2.2
ACKr_c 2.7.2.1;2.7.2.15
MNXR85100_c 1.8.1.2;1.8.1.9
MNXR59708_c 6.1.1.10
MNXR85102_c 4.4.1.16
MNXR61123_c 4.4.1.1;4.4.1.13
MNXR74367_c 4.4.1.8
MNXR75677_c 2.1.1.13;2.1.1.14
MNXR61128_c 1.8.1.9
MNXR35865_c 3.5.5.1
MNXR59296_c 3.5.5.1
MNXR74185_c 2.3.2.2
MNXR22682_c 3.2.1.21
MNXR59575_c 2.3.2.2
MNXR23958_c 3.2.1.21
MNXR12066_c 3.2.1;3.2.1.21
MNXR84958_c 3.2.1.118;3.2.1.21
MNXR8496_c 3.2.1.117;3.2.1.21
UAMAS_c 6.3.2.8
UAMAGS_c 6.3.2.9
GLUR_c 5.1.1.3
MNXR15056_c 2.6.1.21
MNXR15203_c 2.6.1.21
ALAALAr_c 6.3.2.4
ALATA_D_c 2.6.1.21
ALAR_c 5.1.1.1
MNXR74371_c 3.4.11.1;3.4.11.2;3.4.11.23;3.4.13.-;3.4.13.3
MNXR74173_c 2.3.2.2
GTHP_c 1.11.1.9
GTHS_c 6.3.2.3
GLUCYS_c 6.3.2.2
GTHRDHpp_c 3.4.19;3.4.19.13
AMPTASECG_c 3.4.11.1;3.4.11.2;3.4.11.23;3.4.13.-;3.4.13.18
MNXR84567_c 2.3.2.2
MNXR74084_c 2.5.1.18
APCS_c 2.5.1;2.5.1.16
MNXR14507_c 3.2.1.21
SAGH_c 3.2.1.20;3.2.1.26;3.2.1.48
MNXR85086_c 3.2.1.21
UGE_c 5.1.3.6
GLGC_c 2.7.7.27
MNXR59322_c 2.4.1.18
MNXR14508_c 3.2.1.20
MNXR59323_c 2.4.1.1
MNXR59801_c 2.4.1.25
MNXR59368_c 2.4.1.21
UAGDP_c 2.7.7.23
PGAMT_c 5.4.2.10
G1PACT_c 2.3.1.157
G6PDA_c 3.5.99.6
UAGCVT_c 2.5.1.7
MNXR56251_c 1.1.1.158;1.3.1.98
UAPGR_c 1.3.1.98
AHEXASE3_c 3.2.1.52
MI3PP_c 2.7.1.64;3.1.3.25
TDPGDH_c 4.2.1.46
KDOPP_c 3.1.3.45
TDSK_c 2.7.1.130
LPADSS_c 2.4.1.182
GMHEPPA_c 3.1.3.82
MOAT2_c 2.4.99.13
MOAT_c 2.4.99.12
USHD_c 3.6.1.54
KDOPS_c 2.5.1.55
UHGADA_c 3.5.1.108
MNXR9056_c 3.1.3.83
MNXR19003_c 5.3.1.28
MNXR56541_c 3.6.1.27
UAGPT2_c 2.4.1.227
MNXR59903_c 2.7.8.13
UGLDDS2_c 6.3.2.10
MNXR36199_c 2.7.8.13
UAGPT3_c 2.4.1.227
MNXR84924_c 2.3.1.51
MNXR84812_c 2.3.1.15
DHAK_c 2.7.1.29
MNXR1417_c 2.7.1.107
MI4PP_c 3.1.3.25
MI1PP_c 3.1.3.25
GPDDA2_c 3.1.4.2;3.1.4.46
G1PDH_c 1.1.1.8;1.1.1.94
G3PD2_c 1.1.1.94
MNXR76415_c 3.1.3.27
MNXR70333_c 2.7.7.41
MNXR4476_c 4.1.1.65
MNXR84851_c 2.1.1.16;2.1.1.71
GPDDA1_c 3.1.4.2;3.1.4.46
MNXR85074_c 2.1.1.16;2.1.1.71
MNXR84907_c 2.1.1.17
MNXR1099_c 2.7.8.5
MNXR17089_c 1.11.1.9
MNXR60350_c 1.11.1.9
MNXR74164_c 2.3.2.2
PPDK_c 2.7.9.1
MNXR84930_c 2.7.1.40
LGTHL_c 4.4.1.5
LDH_D_c 1.1.1.28
GLYOX_c 3.1.2.6
OAADC_c 1.1.1.38;1.1.1.40;4.1.1.-;4.1.1.3
ME1_c 1.1.1.38;1.1.1.39
MNXR56276_c 6.2.1.1
MNXR56306_c 6.2.1.1
ACCOAC_c 6.4.1.2
ACYP_2_c 3.6.1.7
MNXR6788_c 1.14.12.18
MNXR15995_c 1.14.12.18
MNXR15993_c 1.14.12.18
MNXR15994_c 1.14.12.18
MNXR7592_c 1.14.12.11
MNXR15357_c 1.14.13.-;1.14.13.7
MNXR15072_c 1.14.13.-
MNXR10316_c 1.18.6.1
MNXR15972_c 1.1.1.1
MNXR15973_c 1.1.1.1
MNXR61041_c 1.1.2.7
MNXR75631_c 1.14.12.11
MNXR75632_c 1.14.12.11
MNXR16994_c 1.1.1.1
MNXR12173_c 1.1.1.1
MNXR6332_c 3.1.3.1;3.1.3.2;3.1.3.41
MNXR15996_c 1.14.-.-
MNXR6101_c 1.14.12.1
MNXR5836_c 1.14.12.1
MNXR85646_c 1.14.-.-
MNXR17479_c 1.14.-.-
MNXR17476_c 1.14.-.-
MNXR73770_c 3.6.1.7
MNXR9872_c 3.5.5.1
DDPGA_c 4.1.3.16;4.1.3.42
GLYCTO1_c 1.1.3.15
FDH_c 1.2.1.2
MNXR7109_c 4.1.3.24
MNXR9750_c 6.2.1.9
RBCh_c 4.1.1;4.1.1.39
PGLYCP_c 3.1.3.18
GLYCL_c 1.4.4.2;1.8.1.4;2.1.2.10
MNXR17778_c 1.12.7.2;1.12.99.6;1.2.7.1;1.2.7.4;1.2.99.2
MNXR17785_c 1.-.-.-
MNXR17762_c 1.-.-.-
MNXR8970_c 1.-.-.-
MICITD_c 4.2.1.99
MNXR5630_c 6.2.1.1;6.2.1.17
MNXR5887_c 2.8.3.1;6.2.1.1;6.2.1.17
PPAKr_c 2.7.2.1;2.7.2.15
APLh_c 2.2.1.6
ITCOALm_c 6.2.1.5
MNXR14704_c 4.1.3.24
FGFTh_c 2.1.2.2
DHFR2i_c 1.5.1.3
DHFR_c 1.5.1.3
DHFOR_c 1.5.1.3
FOLR2_c 1.5.1.3
MTHFR3_c 1.5.1.20
MTHFR2_c 1.5.1.20
FMETTRS_c 2.1.2.9
FTHFL_c 6.3.4.3
THFOR1_c 1.5.1.3
THFOR2_c 1.5.1.3
NAD_H2_c 1.12.1.2;1.12.1.5
MNXR84835_c 1.1.2.7
MNXR17799_c 4.2.1.147
MNXR17800_c 1.5.1;1.5.1.-
MNXR5519_c 3.5.4.27
MNXR6072_c 2.3.1.101
MNXR17801_c 1.2.99.5
MNXR6057_c 1.14.13.25
MNXR26440_c 1.2.99.5
MNXR18595_c 2.1.2;2.1.2.1
FBA3_c 4.1.2;4.1.2.13
PRUK_c 2.7.1.19
RBPC_c 4.1.1.39
MNXR76933_c 1.4.3.19
MNXR85599_c 2.8.1.7
MNXR71755_c 4.1.99.17
HMPK1_c 2.7.1.49
TMPPP_c 2.5.1.3
GTPCII_c 3.5.4.25
DHPPDA_c 3.5.4.26
APRAUR_c 1.1.1.193
MNXR18583_c 1.13.11.79
4HTHRS_c 4.2.3;4.2.3.1
MNXR85368_c 1.1.1.262
OHPBAT_c 2.6.1.52
MNXR8716_c 2.6.99.2
NT5C_c 3.1.3.-;3.1.3.5
NNAT_c 2.7.7.1;2.7.7.18
NADS2_c 6.3.5.1
NNAM_c 3.5.1.19
NMNAT_c 2.7.7.1;2.7.7.18
NMNHYD_c 3.1.3.-;3.1.3.5
NADK_c 2.7.1.23
NADTRHD_c 1.6.1.1;1.6.1.2;1.6.1.3
DPCOAK_c 2.7.1.24
APNPT_c 2.7.1.33;2.7.1.34
PPCDC_c 4.1.1.36
APCPT_c 2.7.1.33
PPNCL3_c 6.3.2;6.3.2.5
PPNCL2_c 6.3.2.5
PNTK_c 2.7.1.33
DPR_c 1.1.1.169
MOHMT_c 2.1.2.11
KARA1_c 1.1.1.86
PTPAT_c 2.7.7.3
MNXR85314_c 6.3.4.10;6.3.4.11;6.3.4.15;6.3.4.9
BACCL_c 6.2.1.11;6.3.4.10;6.3.4.11;6.3.4.15;6.3.4.9
MNXR84833_c 2.8.1.6
DBTS_c 6.3.3.3
AMAOTr_c 2.6.1.62
AOXSr_c 2.3.1.47
PMEACPE_c 3.1.1.85
AOXSr2_c 2.3.1.47
MNXR75718_c 2.1.1.197
MNXR75823_c 1.1.1.100
OGMEACPD_c 4.2.1.59
EGMEACPR_c 1.3.1.10
MNXR86014_c 2.3.1.179;2.3.1.41
MNXR21510_c 1.1.1.100
OPMEACPD_c 4.2.1.59
EPMEACPR_c 1.3.1.10
MNXR86013_c 2.3.1.179;2.3.1.41
MNXR85656_c 2.8.1.8
MNXR85657_c 2.3.1.181
MNXR85655_c 2.8.1.8
MNXR85654_c 2.3.1.181
ADCS_c 2.6.1.85
ADCL_c 4.1.3.38
MNXR36005_c 6.3.2.17
DHFS_c 6.3.2.12;6.3.2.17
DHPS_c 2.5.1;2.5.1.15
HPPK_c 2.7.6.3
DHNPA_c 4.1.2.25
MNXR74304_c 3.5.4.16
MNXR74388_c 3.5.4.16
MNXR85297_c 3.5.4.16
MNXR73656_c 3.5.4.16
AKP1_c 3.1.3.1
CPMPS_c 4.1.99.18
MNXR85919_c 2.8.1.12
6CTDS_c 4.1.2.50
MNXR61298_c 4.3.99.3
MNXR19155_c 6.3.4.20
CDGR_c 1.7.1.13
CPPPGO2_c 1.3.99.22
CPPPGO_c 1.3.3.3
UPPDC1_c 4.1.1.37
UPP3S_c 4.2.1.75
UPPDC2_c 4.1.1.37
HMBS_c 2.5.1.61;4.2.1.24
PPBNGS_c 4.2.1.24
G1SAT_c 5.4.3.8
GLUTRR_c 1.2.1.70
GLUTRS_c 6.1.1.17;6.1.1.24
MNXR85382_c 4.99.1.3
MNXR59918_c 2.1.1.151
PC17M_c 2.1.1.131
PC11M_c 2.1.1.133
MNXR34129_c 6.3.5.9
MNXR85384_c 6.3.5.11
MNXR85460_c 2.7.1.156
ACBIPGT_c 2.7.7.62
RZ5PP_c 3.1.3.73
NNDMBRT_c 2.4.2.21
ADCPS2_c 6.3.1.10
CODSCL5BMT_c 2.1.1.195
DXPS_c 2.2.1.7
DXPRIi_c 1.1.1.267
CDPMEK_c 2.7.1.148
MECDPS_c 4.6.1.12
MNXR57061_c 1.17.7.1
DMPPS_syn_c 1.17.1.2
IDS1_c 1.17.1.2
IPDPS_c 1.17.1.2
DMATT_c 2.5.1.1
UDCPDPS_c 2.5.1.31
OCTDPS_c 2.5.1.90
MNXR85991_c 3.4.22.-;3.4.24.84
MNXR85430_c 1.14.-.-
MNXR60109_c 1.14.-.-
PSPPS_c 2.5.1.103;2.5.1.21;2.5.1.96
SS_c 1.3.1.96;2.5.1.21
SQLC_c 5.4.99.17
SQLC2_c 4.2.1.129
MNXR86022_c 1.7.2.6
MNXR84805_c 1.7.99.4
MNXR85317_c 1.18.6.1
MNXR14622_c 3.5.5.1;3.5.5.2;3.5.5.5;3.5.5.7
HCO3E_c 4.2.1.1
MNXR70905_c 1.7.2.5
MNXR85318_c 1.19.6.1
BPNT2_c 3.1.3.7
MNXR68614_c 4.4.1.8
CYSTRS_c 6.1.1.16
PROTRS_c 6.1.1.15
GLNTRAT_c 6.3.5.7
ASNTRAT_c 6.3.5.6
MNXR85732_c 6.1.1.11
FACOAE181_c 3.1.2.2
FACOAE180_c 3.1.2.2
FACOAE160_c 3.1.2.2;3.1.2.22
3OACOAR_c 1.1.1.211;1.1.1.330
COA1819ZD9DS_c 1.14.19.1
MNXR85065_c 1.14.19.2
MNXR4097_c 3.1.3.4;3.1.3.81
MNXR61271_c 2.7.1.174
DORNOp_c 1.4.3.3
GLUN_c 1.4.1.13;1.4.7.1;3.5.1.2;3.5.1.38;4.3.3.6;6.3.4.2;6.3.5.2;6.3.5.4;6.3.5.5
ACONT_c 4.2.1.3
SQLS_c 2.5.1.21
MNXR6078_c 3.5.1.22
ASPO2y_c 1.4.1.21
GAPDH_nadp_hi_c 1.2.1.13;1.2.1.59
MNXR85051_c 1.5.-.-;1.5.1.12;1.5.99.8
MNXR7121_c 1.4.1.20
MNXR10200_c 1.4.3.2
MNXR8072_c 2.6.1.58
PPND2_c 1.3.1.13
ASNS2_c 6.3.1.1;6.3.5.4
ASNN_c 3.5.1.1;3.5.1.38;3.5.5.4
HCYSMT_c 2.1.1.10
BHMT_c 2.1.1.5
MNXR5949_c 1.4.3.2
MNXR5732_c 1.4.1.9
VPAMT_c 2.6.1.66
MNXR14818_c 1.4.1.23;1.4.1.9
MNXR84944_c 1.1.5.8;1.1.99.25
MNXR56274_c 1.1.1;1.1.1.25;1.1.1.282
MNXR84844_c 1.5.5.2
CYSTA_c 2.6.1.1;2.6.1.3
MNXR74373_c 1.3.1.10;1.3.1.104;1.3.1.39;2.3.1.85;2.3.1.86
EAR40y_c 1.3.1.10;1.3.1.104;1.3.1.39;2.3.1.85;2.3.1.86
PRDX_c 1.11.1.21;1.11.1.6;1.11.1.7
ALCD1_c 1.1.1.1;1.1.1.244
MNXR10335_c 1.1.3.13
AICART2_c 6.3.4.23
MNXR6468_c 3.2.2.12
MNXR84884_c 1.3.5.2
DHORD_NAD_c 1.3.1.14
MNXR75060_c 2.7.8.29
NADS1_c 6.3.1.5;6.3.5.1
MNXR59223_c 2.5.1.75;2.5.1.8
MNXR73718_c 2.4.1.83
MNXR74445_c 1.1.2.8
MNXR16109_c 1.14.12.-
MNXR71516_c 2.7.1.161
MNXR8683_c 1.5.7.1
MNXR14750_c 2.1.2.7
RDH1_c 1.1.1.1;1.1.1.105
RDH1a_c 1.1.1.-;1.1.1.300
DPCOAPP_c 3.6.1.9
PDH_c 1.2.1;1.2.4.1;1.8.1.4;2.3.1.12
PFL_c 1.97.1.4;2.3.1.54
MNXR84837_c 1.2.7.1
MNXR8294_c 2.7.2.12
MNXR70855_c 1.4.2.1
GLYOp_c 1.4.3.19;1.4.3.3
GLYCLTDy_c 1.1.1.79
GLYCLTDx_c 1.1.1.26;1.1.1.29;1.1.1.79
ACITL_c 2.3.3.8;4.1.3.6
PC_c 6.4.1.1
MNXR14892_c 3.1.3.58;3.1.3.9
MNXR73876_c 2.7.1.63
MNXR18584_c 2.7.1.147
DADNK_c 2.7.1.145;2.7.1.74;2.7.1.76
DGNSKm_c 2.7.1.113;2.7.1.145;2.7.1.74
NDP6_c 3.6.1.12;3.6.1.6
MNXR73445_c 1.4.3.2
MNXR18726_c 2.6.1.58
SLFAT_c 2.7.7.5
MNXR5457_c 3.6.2.1
ALCD2y_c 1.1.1.1;1.1.1.2;1.1.1.71
MNXR74431_c 1.1.2.8
PPS_c 2.7.9.2
MNXR84814_c 1.8.7.1
MNXR79218_c 1.8.99.1
MNXR9724_c 6.2.1.13
MNXR85228_c 1.5.98.1
ICDHy_c 1.1.1.42
MNXR73685_c 6.2.1.4
AKGDH_c 1.2.4.2;1.8.1.4;2.3.1.61
MNXR84873_c 1.2.4.2
MNXR84917_c 1.3.5.1;1.3.5.4
FBP_c 3.1.3.11
PFK_(adp)_c 2.7.1.146
ALATA_L_c 2.6.1.2
ASPTA6_c 2.6.1.12
MNXR14982_c 1.4.1.9;1.4.9
MOD_3mop_c 1.2.4.4
MNXR85627_c 1.2.4.4
MNXR85034_c 2.3.1.168
CITL_c 4.1.3.6
BDG2HCGHD_c 3.2.1.21
MNXR59619_c 1.14.-.-
MNXR74776_c 2.2.1;2.2.1.1
MNXR73874_c 2.7.1.63
MNXR18585_c 2.7.1.147
CITMCOALm_c 4.1.3.25
MNXR14596_c 2.8.3.22
MNXR6705_c 3.11.1.2
MNXR60478_c 1.14.-.-
PFK_ppi_c 2.7.1.90
MNXR7517_c 1.14.12.3
MNXR26464_c 1.1.99.14
MNXR15981_c 1.13.11;1.14.12.-
MNXR17615_c 3.5.5.1
MNXR6148_c 1.2.1.2;1.2.1.43
MNXR84803_c 1.7.7.2
NITR_c 1.7.1.1;1.7.1.2
MNXR6576_c 1.7.1.2;1.7.1.3
NHFRBO_c 1.7.1.14
MNXR9366_c 1.7.1.14
MNXR75760_c 1.7.1.14
MNXR19023_c 1.7.1.14
MNXR84802_c 1.7.7.1
MNXR70768_c 1.7.2.2
MDHy_c 1.1.1.299;1.1.1.82
MNXR5662_c 1.1.1.85
OMCDC_c 1.1.1.85
MNXR35657_c 1.1.1.42
MNXR5404_c 1.1.1.41;1.1.1.42
MNXR6217_c 4.2.1.33
###Markdown
Save the 'corrected' model as SMBL for further use.
###Code
for rxn in cameo_model.reactions:
if rxn.name == "1 H2O + 1 flavin mononucleotide = 1 riboflavin + 1 phosphate" or rxn.name == "1 H(+) + 1 AMP + 1 D-fructofuranose 1,6-bisphosphate(4-) = 1 ADP + 1 beta-D-fructofuranose 6-phosphate(2-)":
print rxn.id
cameo_model.reactions.get_by_id('ACP1(FMN)_c').id = 'ACP1_FMN_c'
cameo_model.reactions.get_by_id('ACP1(FMN)_c').notes['BIGG'] = ['ACP1_FMN']
cameo_model.repair()
cameo_model.reactions.get_by_id('PFK_(adp)_c').id = 'PFK_adp_c'
cameo_model.reactions.get_by_id('PFK_(adp)_c').notes['BIGG'] = ['PFK_adp']
cameo_model.repair()
cameo_model.compartments['c'] = 'cytosol'
cameo_model.compartments['e'] = 'extracellular'
cameo_model.compartments['bm'] = 'biomass'
for met in cameo_model.metabolites:
if met.id.endswith('_c'):
met.compartment = 'c'
###Output
_____no_output_____
###Markdown
Add Subsystem annotation from KEGG:
###Code
from bioservices import KEGG
k = KEGG()
no_kegg = []
pathway_subsystem_dict = {}
for rxn in cameo_model.reactions:
if 'KEGG' in rxn.notes and rxn.notes['KEGG']:
res = k.get(rxn.notes['KEGG'][0])
pathway_subsystem_dict[rxn] = k.parse(res)
else:
no_kegg.append(rxn)
print len(no_kegg)
subsystem_available = []
no_subsystem = []
no_kegg = []
for rxn in cameo_model.reactions:
if 'KEGG' in rxn.notes and rxn.notes['KEGG'] and type(pathway_subsystem_dict[rxn]) == dict:
d = pathway_subsystem_dict[rxn]
if 'PATHWAY' in d and d['PATHWAY']:
pathway_dict = d['PATHWAY']
subsystem_available.append(rxn)
rxn.subsystem = ",".join(['%s:%s' % (i,j)for i,j in pathway_dict.iteritems()])
else:
no_subsystem.append(rxn)
else:
no_kegg.append(rxn)
print len(subsystem_available), len(no_subsystem)
for rxn in cameo_model.reactions:
print rxn.subsystem
target_filename = relative_directory + '/Reconstructions/MethylococcusModel1.xml'
cobra.io.write_legacy_sbml(cameo_model, target_filename,use_fbc_package=False)
###Output
_____no_output_____
###Markdown
Mapping of the JSON File created with ESCHER
###Code
import json
with open(relative_directory + '/Reconstructions/Metabolic Map/Metanotrophy_genome_scale.json','r') as json_file:
json_data = json.load(json_file)
nodes_json = json_data[1]['nodes']
for key in nodes_json.keys():
if 'bigg_id' in nodes_json[key].keys() and nodes_json[key]['bigg_id']:
content_id = nodes_json[key]['bigg_id']
if content_id.startswith('MNXM'):
content_id = content_id.split('_')
if content_id[0] in chem_xref_MNX_2_dict.keys() and type(chem_xref_MNX_2_dict[content_id[0]]['bigg']) != type(x) and chem_xref_MNX_2_dict[content_id[0]]['bigg'] != []:
bigg_id = chem_xref_MNX_2_dict[content_id[0]]['bigg'][0]
print bigg_id
json_data[1]['nodes'][key]['bigg_id'] = str(bigg_id) +'_'+ content_id[1]
else:
if'name' in nodes_json[key].keys() and nodes_json[key]['name']:
compartment = json_data[1]['nodes'][key]['bigg_id'].split('_')
for met in cameo_model.metabolites:
if met.name == nodes_json[key]['name'] and compartment[1] == met.compartment:
json_data[1]['nodes'][key]['bigg_id'] = met.id
nodes_json = json_data[1]['reactions']
for key in nodes_json.keys():
if 'bigg_id' in nodes_json[key].keys() and nodes_json[key]['bigg_id']:
content_id = nodes_json[key]['bigg_id']
if content_id.startswith('MNXR'):
content_id = content_id.split('_')
if content_id[0] in reac_xref_MNX_2_dict.keys() and type(reac_xref_MNX_2_dict[content_id[0]]['bigg']) != type(x) and reac_xref_MNX_2_dict[content_id[0]]['bigg'] != []:
bigg_id = reac_xref_MNX_2_dict[content_id[0]]['bigg'][0]
print bigg_id
json_data[1]['reactions'][key]['bigg_id'] = str(bigg_id) +'_'+ content_id[1]
else:
if'name' in nodes_json[key].keys() and nodes_json[key]['name']:
for rxn in cameo_model.reactions:
if rxn.name == nodes_json[key]['name']:
print json_data[1]['reactions'][key]['bigg_id']
json_data[1]['reactions'][key]['bigg_id'] = rxn.id
print json_data[1]['reactions'][key]['bigg_id']
with open(relative_directory + '/Reconstructions/Metabolic Map/Metanotrophy_genome_scale_update.json','w') as json_file:
json_file.write(json.dumps(json_data))
###Output
_____no_output_____ |
RobustRegression.ipynb | ###Markdown
Beyond Least Squares Measuring the size of the error with different normsWe define the error as\begin{eqnarray} e = y - Aw\end{eqnarray}Least Squares measures the Euclidian norm of the error \begin{eqnarray} E(w) = \frac{1}{2}e^\top e = \frac{1}{2} \|e\|_2^2\end{eqnarray}here \begin{eqnarray}\|e\|_2 & = & \left(e_1^2 + e_2^2 + \dots + e_N^2\right)^{\frac{1}{2}}\end{eqnarray}Another possibility is measuring the error with other norms, such as the absolute error\begin{eqnarray}\|e\|_1 & = & \left|e_1\right| + \left|e_2\right| + \dots + \left|e_N\right|\end{eqnarray}and more general $p$ norms\begin{eqnarray}\|e\|_p & = & \left( \left|e_1\right|^p + \left|e_2\right|^p + \dots + \left|e_N\right|^p\right)^{\frac{1}{p}}\end{eqnarray} Regularization Measuring the size of the parameter vectorThe idea is introducing a penalty for the parameter values $w$ that are away from the origin$$F_{(2,2)}(w) = \| y - Aw \|_2^2 + \lambda \| w \|_2^2 $$* Lasso penalty $$F_{(2,1)}(w) = \| y - Aw \|_2^2 + \lambda \| w \|_1$$* $\ell_1$ Cost function $$F_{(1,1)}(w) = \| y - Aw \|_1 + \lambda \| w \|_1$$* A genaral mixed norm$$F_{(p,q)}(w) = \| y - Aw \|_p^p + \lambda \| w \|_q^q$$ AsideA norm $\|\cdot\|: \mathbb{C}^m \rightarrow \mathbb{R}$:* (Nonnegativity) $\|x\| \geq 0$, $\|x\| = 0 \Leftrightarrow x = 0$* (Triangle Inequality) $\|x+y\| \leq \|x\| + \| y \|$* (Scaling) $\|\alpha x\| = \left|\alpha\right|\|x\|$ for a scalar $\alpha$, When the cost functions are convex, we can compute the global optimal solution. A general tool for convex optimization is cvx. Measuring the error with different normsBelow example illustrates the effect of choosing a different norms as penalty (cost) functions. Using norms with $p$ close to $1$ has the effect of providing robustness agains outliers.The square function causes large deviations to have an even larger impact on the total error.
###Code
# A toy data set with outliers
x = np.matrix('[0,1,2,3,4,5]').T
y = np.matrix('[2,4,6,-1,10,12]').T
# Degree of the fitted polynomial
degree = 1
N = len(x)
A = np.hstack((np.power(x,i) for i in range(degree+1)))
xx = np.matrix(np.arange(-1,6,0.1)).T
A2 = np.hstack((np.power(xx,i) for i in range(degree+1)))
# Norm parameter
for p in np.arange(1,5,0.5):
# Construct the problem.
w = cvx.Variable(degree+1)
objective = cvx.Minimize(cvx.norm(A*w - y, p))
#constraints = [0 <= x, x <= 10]
#prob = Problem(objective, constraints)
prob = cvx.Problem(objective)
# The optimal objective is returned by prob.solve().
result = prob.solve()
# The optimal value for x is stored in x.value.
print(w.value)
# The optimal Lagrange multiplier for a constraint
# is stored in constraint.dual_value.
#print(constraints[0].dual_value)
plt.figure()
plt.plot(x.T.tolist(), y.T.tolist(), 'o')
plt.plot(xx, A2*w.value, '-')
plt.title('p = '+str(p))
plt.show()
###Output
[[ 2.]
[ 2.]]
###Markdown
Overcomplete Representations and regularizationSuppose we are given a point $y$ in two dimensional space and want to represent this point with a linear combination of $N$ vectors $a_i$ for $i=1\dots N$, where $N>2$. We let $A$ be the $2 \times N$ matrix$$A = [a_1, a_2,\dots, a_N]$$To represent $y$, we need to solve the set of equations$$y = Aw$$Clearly, there could be more than one solution, in fact in general there are an infinite number of solutions $w^*$ to this problem, so minimization of the error is not sufficient.To find a particular solution we may require an additional property from the solution $w^*$, such as having a small norm $\|w\|$. To achieve this, we can try to minimize $$E_{(2,2)}(w) = \| y - Aw \|_2^2 + \lambda \| w \|_2^2 $$
###Code
N = 7
#th = np.arange(0, np.pi-np.pi/N, np.pi/N)
th = 2*np.pi*np.random.rand(N)
A = np.vstack((np.cos(th), np.sin(th)))
y = np.mat('[1.2;2.1]')
fig = plt.figure(figsize=(8,8))
for i in range(len(th)):
plt.arrow(0,0,A[0,i],A[1,i])
plt.plot(y[0],y[1],'ok')
plt.gca().set_xlim((-3,3))
plt.gca().set_ylim((-3,3))
plt.show()
###Output
_____no_output_____
###Markdown
Below, you can experiment by selecting different norms, $$E_{(p,q)}(w) = \| y - Aw \|_p^p + \lambda \| w \|_q^q $$
###Code
## Regularization
lam = 0.02
p = 2
q = 1
def Visualize_Basis(A,w=None, x=None,ylim=[-0.5, 1.1]):
K = A.shape[1]
if x is None:
x = np.arange(0,A.shape[0])
if w is None:
plt.figure(figsize=(6,2*K))
#plt.show()
for i in range(K):
plt.subplot(K,1,i+1)
plt.stem(x,A[:,i])
plt.gcf().gca().set_xlim([-1, K+2])
plt.gcf().gca().set_ylim(ylim)
plt.gcf().gca().axis('off')
plt.show()
else: # if w is not None
plt.figure(figsize=(6,2*K))
for i in range(K):
plt.subplot(K,2,2*i+1)
plt.stem(x,A[:,i])
plt.gcf().gca().set_xlim([-1, K+2])
plt.gcf().gca().set_ylim(ylim)
plt.gcf().gca().axis('off')
plt.subplot(K,2,2*i+2)
plt.stem(x,A[:,i]*w[i])
plt.gcf().gca().set_xlim([-1, K+2])
if np.abs(w[i])<1:
plt.gcf().gca().set_ylim(ylim)
plt.gcf().gca().axis('off')
plt.show()
# Construct the problem.
w = cvx.Variable(len(th))
objective = cvx.Minimize(cvx.norm(A*w - y, p)**p + lam*cvx.norm(w, q)**q)
constraints = []
prob = cvx.Problem(objective, constraints)
# The optimal objective is returned by prob.solve().
result = prob.solve()
figw = 12
fig = plt.figure(figsize=(figw,figw))
ws = np.array(w.value)
v = np.zeros(2)
for i in range(len(th)):
dx = A[0,i]*ws[i]
dy = A[1,i]*ws[i]
plt.arrow(v[0],v[1], dx[0], dy[0],color='red')
v[0] = v[0]+dx
v[1] = v[1]+dy
plt.arrow(0,0, dx[0], dy[0])
plt.arrow(0,0,A[0,i],A[1,i],linestyle=':')
plt.plot(y[0],y[1],'ok')
plt.gca().set_xlim((-1,3))
plt.gca().set_ylim((-1,3))
plt.show()
fig = plt.figure(figsize=(figw,1))
plt.stem(ws, markerfmt='.b', basefmt='b:')
plt.axes().set_xlim((-1,N))
plt.gca().axis('off')
plt.show()
Visualize_Basis(A,w=ws,ylim=[-2,2])
###Output
_____no_output_____
###Markdown
Outlier detection with Basis RegressionSet up some data with outliers
###Code
import scipy as sc
import numpy as np
import pandas as pd
import matplotlib as mpl
import matplotlib.pylab as plt
df_arac = pd.read_csv(u'data/arac.csv',sep=';')
BaseYear = 1995
x = np.matrix(df_arac.Year[31:]).T-BaseYear
y = np.matrix(df_arac.Car[31:]).T/1000000.0
# Introduce some artificial outliers
y[-3] = y[-3]-3
y[4] = y[4]+5
plt.plot(x+BaseYear, y, 'o')
plt.xlabel('Year')
plt.ylabel('Number of Cars (Millions)')
plt.xticks(range(BaseYear, BaseYear+len(x)+3,2))
plt.show()
###Output
_____no_output_____
###Markdown
A model for outlier detectionA design matrix with a union of bases* Smooth low order polynomials for regular behavior $A_{\text smooth}$* Spikes at every time point to model outliers $A_{\text spike}$$$y \approx A_{\text smooth} w_{\text smooth} + A_{\text spike} w_{\text spike}$$* Leads to an interpretable decomposition in terms of actual signal and noise* Write in the generic form $y = Aw$ by$$A = \left[A_{\text smooth}\; A_{\text spike}\right] \left(\begin{array}{c} w_{\text smooth} \\ w_{\text spike}\end{array}\right)$$* Minimize the following objective$$E(w_{\text smooth}, w_{\text spike}) = \|y - A_{\text smooth} w_{\text smooth} - A_{\text spike} w_{\text spike} \|_{2}^2 + \lambda \|w_{\text spike}\|_1$$
###Code
def triag_ones(N):
A = np.zeros((N,N))
for i in range(N):
A[i:,i] = np.ones(N-i)
return A
xx = np.matrix(np.arange(1995,2018,0.5)).T-BaseYear
N = len(x)
degree = 4
B = np.hstack((np.power(x,i) for i in range(degree+1)))
# Make an orthogonal basis that spans the same column space
Q, R, = np.linalg.qr(B)
# Append an extra identity basis for outliers
A = np.hstack((Q, np.eye(N)))
B2 = np.hstack((np.power(xx,i) for i in range(degree+1)))
A2 = B2*R.I
Visualize_Basis(A,x=x)
lam = 0.02
# Construct the problem.
w = cvx.Variable(degree+1+N)
p = 2
q = 1
objective = cvx.Minimize(cvx.norm(A*w - y, p)**p + lam*cvx.norm(w[degree+1:], q)**q)
constraints = []
prob = cvx.Problem(objective, constraints)
# The optimal objective is returned by prob.solve().
result = prob.solve()
plt.figure(figsize=(10,5))
#plt.subplot(2,1,1)
plt.plot(x, y, 'o')
plt.plot(x, A*w.value, 'g:')
plt.plot(xx, A2*w.value[0:degree+1,0], 'r-')
fig.gca().set_xlim((0,25))
plt.show()
fig = plt.figure(figsize=(10,5))
#plt.subplot(2,1,2)
# The optimal value for w is stored in w.value.
plt.stem(x,w.value[degree+1:],basefmt=':')
fig.gca().set_xlim((0,25))
plt.show()
Visualize_Basis(A,x=x,w=np.array(w.value), ylim=[-0.5, 1.1])
###Output
_____no_output_____
###Markdown
Changepoint detection
###Code
x = np.matrix(df_arac.Year[31:]).T-BaseYear
y = np.matrix(df_arac.Truck[31:]).T/1000000.0
plt.plot(x+BaseYear, y, 'o')
plt.xlabel('Year')
plt.ylabel('Number of Trucks(Millions)')
plt.show()
degree = 1
lam = 1
p = 1
q = 1
xx = np.matrix(np.arange(1995,2018,0.5)).T-BaseYear
N = len(x)
B = np.hstack((np.power(x,i) for i in range(degree+1)))
# Make an orthogonal basis that spans the same column space
Q, R, = np.linalg.qr(B)
# Append an extra identity basis for outliers
A = np.hstack((Q, triag_ones(N)))
B2 = np.hstack((np.power(xx,i) for i in range(degree+1)))
A2 = B2*R.I
# Construct the problem.
w = cvx.Variable(degree+1+N)
objective = cvx.Minimize(cvx.norm(A*w - y, p)**p + lam*cvx.norm(w[degree+1:], q)**q)
constraints = []
prob = cvx.Problem(objective, constraints)
# The optimal objective is returned by prob.solve().
result = prob.solve()
plt.figure(figsize=(10,5))
#plt.subplot(2,1,1)
plt.plot(x, y, 'o')
plt.plot(x, A*w.value, ':')
plt.plot(xx, A2*w.value[0:degree+1,0], '-')
fig.gca().set_xlim((0,25))
plt.show()
fig = plt.figure(figsize=(10,5))
#plt.subplot(2,1,2)
# The optimal value for w is stored in w.value.
plt.stem(x,w.value[degree+1:])
fig.gca().set_xlim((0,25))
# Visualize the Basis
K = A.shape[1]
plt.show()
plt.figure(figsize=(6,2*K))
for i in range(K):
plt.subplot(K,2,2*i+1)
plt.stem(x,A[:,i])
plt.gcf().gca().set_xlim([0, K+2])
plt.gcf().gca().set_ylim([-0.5, 1.1])
plt.gcf().gca().axis('off')
plt.subplot(K,2,2*i+2)
plt.stem(x,A[:,i]*w.value[i,0])
plt.gcf().gca().set_xlim([0, K+2])
if np.abs(w.value[i,0])<1:
plt.gcf().gca().set_ylim([-0.5, 1.1])
plt.gcf().gca().axis('off')
plt.show()
%run plot_normballs.py
###Output
_____no_output_____
###Markdown
Feature selection
###Code
p = 2
q = 1
lam = 0.1
K = 200
N = 100
R = 10
w_true = np.zeros(K)
idx = np.random.choice(range(K), size=R)
w_true[idx] = 2*np.random.randn(K,1)
A = np.random.randn(N, K)
y = 0.0*np.random.randn(N) + A.dot(w_true)
# Construct the problem.
w = cvx.Variable(K,1)
objective = cvx.Minimize(cvx.norm(A*w - y, p)**p + lam*cvx.norm(w, q)**q)
prob = cvx.Problem(objective, constraints)
# The optimal objective is returned by prob.solve().
result = prob.solve()
plt.stem(w.value)
plt.stem(range(K),w_true.T,':',markerfmt='wo')
plt.show()
#print w.value
#print w_true
###Output
_____no_output_____
###Markdown
Well log data
###Code
import pandas as pd
lam = 1.2
p = 2
q = 1
df_welllog = pd.read_csv(u'data/well-log.csv',names=['y'])
y = np.array(df_welllog.y)[::4]/100000.
N = len(y)
A = triag_ones(N)
K = N
# Construct the problem.
w = cvx.Variable(K,1)
objective = cvx.Minimize(cvx.norm(A*w - y, p)**p + lam*cvx.norm(w, q)**q)
prob = cvx.Problem(objective, constraints)
# The optimal objective is returned by prob.solve().
result = prob.solve()
thr = 0.01
plt.figure(figsize=(12,4))
plt.plot(A.dot(w.value),'r')
plt.plot(y)
plt.xlim((-5,N))
plt.figure(figsize=(12,4))
idx = np.where(np.abs(w.value)>thr)[0]
plt.stem(idx,w.value[idx])
plt.xlim((-5,N))
plt.show()
###Output
_____no_output_____ |
03 - pyplot/0301 - Introduction to pyplot.ipynb | ###Markdown
In a previous lesson we discussed the three interfaces of matplotlib, and of the two officially supported interfaces, the `pyplot` interface is the most common way to interact with the library. In this lesson, we'll cover some of the basics of the `pyplot` interface and see a few examples of it in action. Importing the InterfaceRather than talk at length about the `pyplot` interface, let's just go ahead and jump right in and start playing around with it. The very first thing you'll want to do is set the notebook up for showing matplotlib output inline. The very first line of code below shows how to do this by calling the `%matplotlib` magic function and passing in the term `'inline'`.
###Code
%matplotlib inline
###Output
_____no_output_____
###Markdown
Following that, you'll want to import the `pyplot` module, and if you remember from an earlier lesson, I mentioned that pretty much every module you'll use in matplotlib, and the scientific python stack for that matter, has an agreed upon way to import it. Line two shows the canonical way to import the `pyplot` module.
###Code
import matplotlib.pyplot as plt
###Output
_____no_output_____
###Markdown
Finally, if you're following along on the command line, you'll need to call the `pyplot.ion()` function that you see in the next cell. Don't worry about what it does just yet, we'll learn about that in just a bit, but for now go ahead and call it so you can follow along with the rest of the tutorial.
###Code
# This does nothing after calling %matplotlib inline,
# but it turns on interactive mode in the command line.
plt.ion()
###Output
_____no_output_____
###Markdown
NOTE: The code in the next cell is only needed if you're running the code on a retina-enabled, or for you non-Mac users, a high PPI display.
###Code
# Turn on retina mode
from IPython.display import set_matplotlib_formats
set_matplotlib_formats('retina')
###Output
_____no_output_____
###Markdown
Understanding the `pyplot` InterfaceBefore we go any further, there are a few things that you need to understand about the `pyplot` interface that will make working with matplotlib a lot smoother. First, the `pyplot` interface is a stateful interface, and second, it has two modes: interactive and non-interactive mode. A Stateful InterfaceNow, what do I mean when I say that `pyplot` provides a "stateful interface"? Well, when you create a visualization there are usually several steps you need to work through to get it just right. For example, you'll need to plot the data itself. Then, you may need to adjust the limits of the axes, and possibly change the labels for the tick marks as well. To make the visualization easier to understand, you may want to add x- and y-axis labels, a title, and maybe even a legend. Doing all of these modifications in one command would be tough enough from a script, but in an interactive interpreter session, like this one, it would be simply too painful to even bother with. Instead, a better way to do this would be to perform each change in a seperate step allowing you to focus on one task at a time. The `pyplot` interface does exactly that through its "stateful interface". In short, every `pyplot` function you call changes the internal state of the current visualization. So, a call to the `plot()` function, for example, may create several objects in the background, or simply update existing ones that were created by a previous function call. The point is, you don't have to worry about creating instances of classes or modifying them directly, instead you can just focus on the visualization. Let's give the stateful interface a try now by building up a simple plot. We'll start by plotting a few randomly generated lines, then we'll add a title to our plot to make it a bit easier to understand what it's displaying. We'll do this in two separate steps to show off the stateful nature of the `pyplot` interface.So, first things first, let's import the numpy library to give us access to some nice functions for generating random data.
###Code
import numpy as np
###Output
_____no_output_____
###Markdown
Now, we can create our plot. First, we'll create a `for` loop, and at each iteration, we'll plot some randomly generated, normally distributed data by calling the `numpy.random.randn` function. After you plot your data, call the `pyplot.title` function to add the title "Normally Distributed Random Samples" to the plot.
###Code
# Plot 3 randomly generated lines
for i in range(3):
plt.plot(np.random.randn(10))
# Add a title to the plot
plt.title('Normally Distributed Random Samples');
###Output
_____no_output_____
###Markdown
The main thing to notice here is that we had four different interactions with the `pyplot` module: 3 calls to the `plot()` function and 1 to the `title()` function, and in each case, the result was that the current visualization was updated with the requested change. The components of that visualization, i.e., the class instances that make up the visualization, are completely invisible to us. Instead, we simply concentrate on the how the visualization should look and ignore everything that goes into making that visualization. This ends up being such an intuitive interface, and it's easy to see why this is the preferred method for interactive data visualization with matplotlib. You essentially lower your cognitive load by concentrating on only one aspect of a visualization at a time and build it up step-by-step. Interactive ModeNow, if you're working from the command line, you may have noticed that the very first call to the `plot` function caused a new figure to pop up in a separate window. The subsequent call to the `title` function actually updated the already existing figure like magic, right in front of your eyes. That's because the call you made earlier to the `ion` function turned on `pyplot's` interactive mode. In interactive mode, every call you make to the `pyplot` module results in a change to the currently displayed figure. Without interactive mode turned on, you would need to call the `pyplot.show` function to display the current figure, but you would lose the ability to interact with that figure once you did. You can give it a try now by first calling the `pyplot.ioff` function to turn off interactive mode.
###Code
plt.ioff()
###Output
_____no_output_____
###Markdown
Incidentally, you can always check if you're currently in interactive mode by calling the `plt.isinteractive()` function. Let's try it out now.
###Code
plt.isinteractive()
###Output
_____no_output_____
###Markdown
Now that we've turned off interactive mode, we can make any number of calls to the `pyplot` interface, and you won't see any output until you call the `pyplot.show` function. Let's give it a try now by plotting a histogram of some randomly generated data.
###Code
plt.hist(np.random.randn(1000));
###Output
_____no_output_____
###Markdown
If you're following along from the command line, you should no longer be seeing a figure pop up when you ran the last line of code. However, for those of you following along in a Jupyter notebook, you may have noticed that a histogram plot appeared as soon as you executed the previous cell. Unfortunately, turning off interactive mode in a notebook is not as easy as just calling the `pyplot.ioff` function. The reason is that our earlier call to the `%matplotlib inline` magic function does a little bit of extra setup for us to get interactive mode working properly in a notebook. So, to turn off interactive mode in this case, we'll need to undo that extra setup. Specifically, an event listener was added to the `'post_execute'` event that will flush the current figure every time we execute the code in a cell. To remove the event listener, we'll first need to grab a reference to the current IPython shell (the one we're currently interacting with), and then we'll remove the `flush_figures` function from the `'post_execute'` event listener for the current shell. So, let's get started by first getting a reference to the current shell. To do so, you can simply call the `get_ipython()` function.
###Code
# Get a reference to the current IPython shell
shell = get_ipython()
###Output
_____no_output_____
###Markdown
Once you have a reference to the current IPython shell, you can call the `unregister` function on the `events` object and pass in the name of the event, in our case that'll be the `'post_execute'` event, followed by a reference to the event handler that we want to remove from the listener, which will be the `flush_figures` function. To do this, we'll first need to import the `flush_figures` function.
###Code
# Import the event handler function that we are trying to unregister
from ipykernel.pylab.backend_inline import flush_figures
###Output
_____no_output_____
###Markdown
Then, we can remove the `flush_figures` function from the list of callback functions registered with the `post_execute` event listener. To do that, we simply call the `unregister` function and pass in the event name and function reference.
###Code
# Unregister the event handler for the current shell session
shell.events.unregister('post_execute', flush_figures)
###Output
_____no_output_____
###Markdown
Now, we should be able to call the `pyplot.hist` function again without displaying anything.
###Code
plt.hist(np.random.randn(1000));
###Output
_____no_output_____
###Markdown
To show our plot now, we'll need to call the `pyplot.show` function, so let's go ahead and do that now.
###Code
plt.show()
###Output
_____no_output_____ |
notebooks/download label files.ipynb | ###Markdown
Table of Contents
###Code
from planet4 import region_data
from hirise_tools.downloads import get_rdr_color_label, download_RED_product
import hirise_tools as ht
# regions = ['Giza', 'Ithaca', 'Manhattan2', 'Inca']
# regions.remove('Inca')
regions = ['Potsdam']
seasons = ['season2', 'season3']
get_rdr_color_label(obsid)
root = '/Volumes/Data/hirise/p4_input'
from nbtools import execute_in_parallel
for region in regions:
print(region)
for season in seasons:
print(season)
reg = getattr(region_data, region)
seas = getattr(reg, season)
execute_in_parallel(get_rdr_color_label, seas)
def get_p4_hirise_data(obsid):
from hirise_tools.downloads import download_RED_product
root = '/Volumes/Data/hirise/p4_input'
for ccdno in [4,5]:
for channel in [0, 1]:
download_RED_product(obsid, ccdno, channel, saveroot=root)
from nbtools import display_multi_progress
for region in regions:
print(region)
for season in seasons:
print(season)
reg = getattr(region_data, region)
seas = getattr(reg, season)
lbview.map_async(get_p4_hirise_data, seas)
display_multi_progress?
for region in regions:
print(region)
for season in seasons:
print(season)
reg = getattr(region_data, region)
seas = getattr(reg, season)
print(sorted(seas))
###Output
_____no_output_____ |
10_MNIST Digits Classification (LeNet).ipynb | ###Markdown
DLVC 2017 Tutorial 10: MNIST Digits Classification (LeNet) MNIST database (http://yann.lecun.com/exdb/mnist/)
###Code
%matplotlib inline
import os
import struct
import torch
from PIL import Image
import matplotlib.pyplot as plt
import numpy as np
import torchvision
from torch.autograd import Variable
from torch.utils.data import TensorDataset,DataLoader
from torchvision import datasets,transforms
import torch.nn as nn
import torch.nn.functional as F
import torch.optim as optim
import copy
import time
# Check availability of GPU
use_gpu = torch.cuda.is_available()
if use_gpu:
pinMem = True # Flag for pinning GPU memory
print('GPU is available!')
else:
pinMem = False
###Output
_____no_output_____
###Markdown
Downloading datset
###Code
apply_transform = transforms.Compose([transforms.Resize(32),transforms.ToTensor()])
trainLoader = torch.utils.data.DataLoader(datasets.MNIST('./MNIST/', train=True, download=True,
transform = apply_transform), batch_size=1024, shuffle=True, num_workers=1, pin_memory=pinMem)
testLoader = torch.utils.data.DataLoader(datasets.MNIST('./MNIST/', train=False,transform=apply_transform),
batch_size=1024, shuffle=True, num_workers=1, pin_memory=pinMem)
# Size of train and test datasets
print('No. of samples in train set: '+str(len(trainLoader.dataset)))
print('No. of samples in test set: '+str(len(testLoader.dataset)))
###Output
_____no_output_____
###Markdown
Define network architecture
###Code
class LeNet(nn.Module):
def __init__(self):
super(LeNet, self).__init__()
self.conv1 = nn.Conv2d(1, 6, kernel_size=5)
self.pool1 = nn.MaxPool2d(kernel_size=2,stride=2)
self.conv2 = nn.Conv2d(6, 16, kernel_size=5)
self.pool2 = nn.MaxPool2d(kernel_size=2,stride=2)
self.conv2_drop = nn.Dropout2d()
self.fc1 = nn.Linear(400, 120)
self.fc2 = nn.Linear(120, 84)
self.fc3 = nn.Linear(84, 10)
def forward(self, x):
x = F.relu(self.conv1(x))
x = self.pool1(x)
x = F.relu(self.conv2_drop(self.conv2(x)))
x = self.pool2(x)
x = x.view(-1, 400)
x = F.relu(self.fc1(x))
x = F.dropout(x, training=self.training)
x = F.relu(self.fc2(x))
x = F.dropout(x, training=self.training)
x = self.fc3(x)
return F.log_softmax(x)
###Output
_____no_output_____
###Markdown
Initialize the network
###Code
net = LeNet()
print(net)
if use_gpu:
net = net.cuda()
###Output
_____no_output_____
###Markdown
Total number of trainable parameters
###Code
totalParams = 0
for params in net.parameters():
print params.size()
totalParams += np.sum(np.prod(params.size()))
print('Total number of parameters: '+str(totalParams))
init_conv1 = copy.deepcopy(net.conv1.weight.data)
###Output
_____no_output_____
###Markdown
Define loss function and optimizer
###Code
criterion = nn.NLLLoss() # Negative Log-likelihood
optimizer = optim.SGD(net.parameters(), lr=1e-2, momentum=0.9) # Stochastic gradient descent with momentum
###Output
_____no_output_____
###Markdown
Train the network
###Code
iterations = 20
trainLoss = []
testAcc = []
start = time.time()
for epoch in range(iterations):
epochStart = time.time()
runningLoss = 0
net.train(True) # For training
for data in trainLoader:
inputs,labels = data
# Wrap them in Variable
if use_gpu:
inputs, labels = Variable(inputs.cuda()), \
Variable(labels.cuda())
else:
inputs, labels = Variable(inputs), Variable(labels)
# Initialize gradients to zero
optimizer.zero_grad()
# Feed-forward input data through the network
outputs = net(inputs)
# Compute loss/error
loss = criterion(outputs, labels)
# Backpropagate loss and compute gradients
loss.backward()
# Update the network parameters
optimizer.step()
# Accumulate loss per batch
runningLoss += loss.data[0]
avgTrainLoss = runningLoss/60000.0
trainLoss.append(avgTrainLoss)
# Evaluating performance on test set for each epoch
net.train(False) # For testing [Affects batch-norm and dropout layers (if any)]
running_correct = 0
for data in testLoader:
inputs,labels = data
# Wrap them in Variable
if use_gpu:
inputs = Variable(inputs.cuda())
outputs = net(inputs)
_, predicted = torch.max(outputs.data, 1)
predicted = predicted.cpu()
else:
inputs = Variable(inputs)
outputs = net(inputs)
_, predicted = torch.max(outputs.data, 1)
running_correct += (predicted == labels).sum()
avgTestAcc = running_correct/10000.0
testAcc.append(avgTestAcc)
# Plotting training loss vs Epochs
fig1 = plt.figure(1)
plt.plot(range(epoch+1),trainLoss,'r-',label='train')
if epoch==0:
plt.legend(loc='upper left')
plt.xlabel('Epochs')
plt.ylabel('Training loss')
# Plotting testing accuracy vs Epochs
fig2 = plt.figure(2)
plt.plot(range(epoch+1),testAcc,'g-',label='test')
if epoch==0:
plt.legend(loc='upper left')
plt.xlabel('Epochs')
plt.ylabel('Testing accuracy')
epochEnd = time.time()-epochStart
print('Iteration: {:.0f} /{:.0f} ; Training Loss: {:.6f} ; Testing Acc: {:.3f} ; Time consumed: {:.0f}m {:.0f}s '\
.format(epoch + 1,iterations,avgTrainLoss,avgTestAcc*100,epochEnd//60,epochEnd%60))
end = time.time()-start
print('Training completed in {:.0f}m {:.0f}s'.format(end//60,end%60))
###Output
_____no_output_____
###Markdown
Visualizing the kernels
###Code
# functions to show an image
def imshow(img, strlabel):
npimg = img.numpy()
npimg = np.abs(npimg)
fig_size = plt.rcParams["figure.figsize"]
fig_size[0] = 10
fig_size[1] = 10
plt.rcParams["figure.figsize"] = fig_size
plt.figure()
plt.title(strlabel)
plt.imshow(np.transpose(npimg, (1, 2, 0)))
trained_conv1 = net.conv1.weight.data
imshow(torchvision.utils.make_grid(init_conv1,nrow=5,normalize=True),'Initial Weights')
imshow(torchvision.utils.make_grid(trained_conv1,nrow=5,normalize=True),'Trained Weights')
###Output
_____no_output_____
###Markdown
Saving the trained model
###Code
torch.save(net.state_dict(), 'trainedNet.pt') # Saving the trained parameters
###Output
_____no_output_____
###Markdown
Loading saved model
###Code
new_net = LeNet()
new_net.load_state_dict(torch.load('trainedNet.pt'))
###Output
_____no_output_____ |
9-14.ipynb | ###Markdown
函数- 函数可以用来定义可重复代码,组织和简化- 一般来说一个函数在实际开发中为一个小功能- 一个类为一个大功能- 同样函数的长度不要超过一屏 定义一个函数def function_name(list of parameters): do something- 以前使用的random 或者range 或者print.. 其实都是函数或者类
###Code
def pd():
num = eval(input('>>'))
if num % 2 == 0:
print (str(num) + '是偶数')
else:
print (str(num) + '是奇数')
pd()
def sushu():
n = eval(input('>>'))
if n % 1 ==0 and n % n ==0:
print ((str(n) + '是素数')
else:
print ((str(n) + '不是素数')
sushu()
###Output
_____no_output_____
###Markdown
调用一个函数- functionName()- "()" 就代表调用  带返回值和不带返回值的函数- return 返回的内容- return 返回多个值- 一般情况下,在多个函数协同完成一个功能的时候,那么将会有返回值 - 当然也可以自定义返回None EP: 类型和关键字参数- 普通参数- 多个参数- 默认值参数- 不定长参数
###Code
def hanshu(x):
y = x**2
print (y)
hanshu(x = 2)
def y(x):
return x**2
y_ = y(100)
print (y_)
def input_():
num = eval(input('>>'))
res3 = san(num)
res2 = liang(num)
print (res3 - res2)
def san(num):
return num**3
def liang(num):
return num**2
input_()
###Output
>>10
900
###Markdown
普通参数 多个参数 默认值参数
###Code
zhanghu = '[email protected]'
mima = '123hahaha'
is_ok_and_y = False
def login(account,password):
if account == zhanghu and password == mima:
print ('登陆成功')
else:
print ('账号或密码错误')
login(account='[email protected]',password='123hahaha')
def qidong():
global is_ok_and_y
if is_ok_and_y ==False:
print('是否七天免登陆')
res = input('>>')
account = input('请输入账号')
password = input('请输入密码')
if res =='y':
login(account,password)
is_ok_and_y = True
else:
login(account,password)
else:
print ('登陆成功')
qidong()
###Output
登陆成功
###Markdown
强制命名 不定长参数- \*args> - 不定长,来多少装多少,不装也是可以的 - 返回的数据类型是元组 - args 名字是可以修改的,只是我们约定俗成的是args- \**kwargs > - 返回的字典 - 输入的一定要是表达式(键值对)- name,\*args,name2,\**kwargs 使用参数名 变量的作用域- 局部变量 local- 全局变量 global- globals 函数返回一个全局变量的字典,包括所有导入的变量- locals() 函数会以字典类型返回当前位置的全部局部变量。 注意:- global :在进行赋值操作的时候需要声明- 官方解释:This is because when you make an assignment to a variable in a scope, that variable becomes local to that scope and shadows any similarly named variable in the outer scope.-  Homework- 1
###Code
def getPentagonalNumber():
count = 0
for n in range(1,101):
result = n*(3*n-1)/2
count = count+1
print (int(result),end=' ')
if count%10 == 0:
print('\n')
getPentagonalNumber()
###Output
1 5 12 22 35 51 70 92 117 145
176 210 247 287 330 376 425 477 532 590
651 715 782 852 925 1001 1080 1162 1247 1335
1426 1520 1617 1717 1820 1926 2035 2147 2262 2380
2501 2625 2752 2882 3015 3151 3290 3432 3577 3725
3876 4030 4187 4347 4510 4676 4845 5017 5192 5370
5551 5735 5922 6112 6305 6501 6700 6902 7107 7315
7526 7740 7957 8177 8400 8626 8855 9087 9322 9560
9801 10045 10292 10542 10795 11051 11310 11572 11837 12105
12376 12650 12927 13207 13490 13776 14065 14357 14652 14950
###Markdown
- 2
###Code
def sumDigits():
x = eval(input('请输入一个三位数:'))
a = x%10
b = x//10
c = b%10
d = b//10
y = a+c+d
print(y)
sumDigits()
###Output
请输入一个三位数:234
9
###Markdown
- 3
###Code
def displaySortedNumbers():
a,b,c = eval(input('请输入三个整数'))
if a>=b and a>=c:
if b>=c:
print (str(a)+ '>' +str(b) + '>' + str(c))
else:
print (str(a)+ '>' +str(c) + '>' + str(b))
elif b>=a and b>=c:
if a>=c:
print (str(b)+ '>' +str(a) + '>' + str(c))
else:
print (str(b)+ '>' +str(c) + '>' + str(a))
elif c>=a and c>=b:
if a>=b:
print (str(c)+ '>' +str(a) + '>' + str(b))
else:
print (str(c)+ '>' +str(b) + '>' + str(a))
displaySortedNumbers()
###Output
请输入三个整数3,2.4,5
5>3>2.4
###Markdown
- 4
###Code
def futureInvestmentValue(investmentAmount,monthlyInterestRate,years=1):
money=investmentAmount
month=years*12
rate=monthlyInterestRate/12
fmoney=money*((1+rate)**month)
return fmoney
money1=eval(input('请输入本金:'))
rate1=eval(input('请输入年利率:'))
years1=1
print('\n')
print('Years Future Value')
while years1 <=30:
futuervalue=futureInvestmentValue(money1,rate1/100,years1)
years1 += 1
print(str(years1-1)+' '+str(round(futuervalue,2)))
###Output
请输入本金:1000
请输入年利率:9
Years Future Value
1 1093.81
2 1196.41
3 1308.65
4 1431.41
5 1565.68
6 1712.55
7 1873.2
8 2048.92
9 2241.12
10 2451.36
11 2681.31
12 2932.84
13 3207.96
14 3508.89
15 3838.04
16 4198.08
17 4591.89
18 5022.64
19 5493.8
20 6009.15
21 6572.85
22 7189.43
23 7863.85
24 8601.53
25 9408.41
26 10290.99
27 11256.35
28 12312.28
29 13467.25
30 14730.58
###Markdown
- 5 - 6
###Code
def numberOfDaysInYear():
for year in range(2010,2021):
if (year % 4 == 0 and year % 100 != 0) or (year % 400 == 0):
print(year,'年有366天')
else:
print(year,'年有365天')
numberOfDaysInYear()
###Output
2010 年有365天
2011 年有365天
2012 年有366天
2013 年有365天
2014 年有365天
2015 年有365天
2016 年有366天
2017 年有365天
2018 年有365天
2019 年有365天
2020 年有366天
###Markdown
- 7
###Code
def distance(x1,y1,x2,y2):
a=x1
b=x2
c=y1
d=y2
nums=((a-b)*(a-b) + (c-d)*(c-d))**0.5
return nums
X1,Y1=eval(input('请输入第一个点的坐标: '))
X2,Y2=eval(input('请输入第二个点的坐标: '))
length=distance(X1,Y1,X2,Y2)
print('两点之间的距离为: '+str(length))
###Output
请输入第一个点的坐标: 2,3
请输入第二个点的坐标: 3,2
两点之间的距离为: 1.4142135623730951
###Markdown
- 8
###Code
def meisen(p):
number = 2**p-1
return number
print('p 2^p-1')
for i in range(2,32):
for j in range(2,i):
if i % j ==0:
break
else:
numbers=meisen(i)
print(str(i)+' '+str(numbers))
###Output
p 2^p-1
2 3
3 7
5 31
7 127
11 2047
13 8191
17 131071
19 524287
23 8388607
29 536870911
31 2147483647
|
notebooks/04_countcorrect_getCorrectedDataMatrix_forCPU.ipynb | ###Markdown
Script gets background corrected data using the naive method or countcorrect with a small number of iterations suitable for cpu work.
###Code
import sys,os
import pickle
import anndata
import pandas as pd
import numpy as np
import scanpy as sc
import matplotlib.pyplot as plt
import seaborn as sns
data_type = 'float32'
os.environ["THEANO_FLAGS"] = 'device=cuda,floatX=' + data_type + ',force_device=True' + ',dnn.enabled=False'
import countcorrect as cc
adata_wta = sc.read_h5ad(open("/lustre/scratch117/cellgen/team283/Kidney-Nanostring/Kidney_AnnData_2.h5ad", "rb"))
counts_geneProbes = np.asarray(adata_wta.X)
counts_negativeProbes = np.asarray(adata_wta.obsm['negProbes'])
counts_nuclei = np.asarray(adata_wta.obs['AOINucleiCount']).reshape(len(adata_wta.obs['AOINucleiCount']),1).squeeze()
X_corrected = cc.run_countcorrect(counts_geneProbes, counts_negativeProbes, counts_nuclei, naive = True)
adata_wta.layers['X_naive'] = X_corrected
X_corrected = cc.run_countcorrect(counts_geneProbes, counts_negativeProbes, counts_nuclei, total_iterations = 1000)
adata_wta.layers['X_corrected_cpu'] = X_corrected
adata_wta.write_h5ad('/lustre/scratch117/cellgen/team283/Kidney-Nanostring/Kidney_AnnData_3.h5ad')
###Output
_____no_output_____ |
lead_generation.ipynb | ###Markdown
Companies House data set used as a list of source companies which could be of interest for B2B lead generation.Obtain the data set here:http://download.companieshouse.gov.uk/en_output.htmlFirst we read the data set into a Pandas DataFrame and serialise it into a pickle file.
###Code
rootdir="/home/ilan/Desktop/GI_interview_project"
datadir="/home/ilan/Desktop/GI_interview_project/company_data"
os.chdir(datadir)
pklfile="data.pkl"
#hffile="data.h5"
folderpath=os.path.join(datadir,pklfile)
#folderpath=os.path.join(rootdir,hffile)
if (os.path.exists(folderpath)==True):
print("Pickle file containing data found. Loading it...")
data=pickle.load(open(folderpath,'r'))
#data = tables.open_file(folderpath, driver="H5FD_CORE")
else:
print("Reading in csv file and creating pickle...")
filenames =['BasicCompanyData-2015-05-01-part1_5.csv', 'BasicCompanyData-2015-05-01-part2_5.csv',\
'BasicCompanyData-2015-05-01-part3_5.csv', 'BasicCompanyData-2015-05-01-part4_5.csv',\
'BasicCompanyData-2015-05-01-part5_5.csv']
list_ = []
# for i,j in enumerate(filenames):
# if (i == 0):
# data = pan.read_csv(j, delimiter=',',index_col=False)
# list_.append(data)
# print data.head(1)
# elif (i > 0):
# data = pan.read_csv(j, delimiter=',',skiprows=1,index_col=False)
# list_.append(data)
# print data.head(1)
# data = pan.concat(list_)
for i in filenames:
data = pan.read_csv(i, delimiter=',',index_col=False)
list_.append(data)
#print data.head(1)
data = pan.concat(list_)
# Remove dots and whitespaces from column titles
colnames = [str(i).replace('.','_').strip() for i in list(data.columns.values)]
data.columns=colnames
# Remove period in the label column
#data['Label']=data['Label'].apply(lambda x: x.strip('.'))
with open(pklfile,'wb') as output:
pickle.dump(data, output, pickle.HIGHEST_PROTOCOL)
os.chdir(rootdir)
data
###Output
Pickle file containing data found. Loading it...
###Markdown
To get a feel for the data set we do some basic data set exploration.
###Code
print data.columns
print data.size
data.describe()
# All the labels in the data, and their counts
categorycounts=data['CompanyCategory'].value_counts()
print categorycounts
categorycounts.plot(kind='bar')
# All the labels in the data, and their counts
statuscounts=data['CompanyStatus'].value_counts()
print statuscounts
statuscounts.plot(kind='bar')
class Mask(object):
def __init__(self,df,field,match):
self.df = df
self.field = field
self.match = match
self.function = lambda x, y, z: x.loc[x[y] == z]
def __call__(self):
return self.function(self.df,self.field,self.match)
#return self.df.loc[self.df[self.field] == self.match]
#data[data.CompanyName == "! LTD"]
#data.loc[data["CompanyName"] == "! LTD"]
result = Mask(data, "CompanyName", "! LTD")
print result()
class booleanMask(object):
def __init__(self,function):
self.function = function
#def __and__(self,other):
# self.function = self.function & other.function
def __call__(self,df):
self.df = df
return map(self.function, [self.df])[0]
company_mask = booleanMask(lambda x: x.CompanyName == "! LTD")
##print company_mask(data)
print data[company_mask(data)]
# MASKS CAN NOW BE COMBINED
#uk_mask = booleanMask(lambda x: x.RegAddress_Country == "UNITED KINGDOM")
#active_mask = booleanMask(lambda x: x.CompanyStatus == "Active")
#print data[uk_mask(data) & active_mask(data)]
# FOR VALIDATION TO MAKE SURE BOOLEANMASK IS GIVING WHAT WE EXPECT
#data.loc[(data["RegAddress_Country"] == "UNITED KINGDOM") & (data["CompanyStatus"] == "Active")]
#print len(data.loc[(data["RegAddress_Country"] == "UNITED KINGDOM") & (data["CompanyStatus"] == "Active")])
#print len(data[uk_mask(data) & active_mask(data)])
#print map(lambda x: x.CompanyName == "! LTD", [data])
# DEFINE A REDUCED DATASET FOR PROTOTYPING
from random import sample
# number/fraction of entries to use
#ents = int(len(X)*0.1)
ents = 100
# Take a random sample from the data
smalldataind = sample(range(0,len(data)-1),ents)
#print smalldataind
# HERE
#smalldataind = [784400, 333248, 3037529, 333413, 1851904, 1569996, 2958604, 769824, 2848095, 896580]
smalldata = data.iloc[smalldataind]
smalldata
# svn checkout http://pygoogle.googlecode.com/svn/trunk pygoogle-read-only
# python setup.py build
# sudo python setup.py install
from pygoogle import pygoogle
from time import sleep
from pprint import pprint
#g = pygoogle('! LTD company')
#g.pages = 1
#print '*Found %s results*'%(g.get_result_count())
#g.get_urls()
#print list(smalldata['CompanyName'].values)
compnames = list(smalldata['CompanyName'].values)
#compadds = list(smalldata['RegAddress_AddressLine1'].values)
compadds = list(smalldata['RegAddress_PostCode'].values)
#compadds = [i.split(' ')[0] for i in list(smalldata['RegAddress_PostCode'].values)]
#urls = []
#counter = 0
#for i,j in zip(compnames,compadds):
# g = pygoogle(i+' contact '+j)
# g.pages = 1
# urls.append(g.get_urls())
# counter += 1
# sleep(np.random.uniform(5,10))
#print urls
os.chdir(datadir)
urlpklfile="URLs.pkl"
urlfolderpath=os.path.join(datadir,urlpklfile)
if (os.path.exists(urlfolderpath)==True):
print("Pickle file containing URL data found. Loading it...")
urls=pickle.load(open(urlfolderpath,'r'))
else:
print("Fetching company URLs from Google...")
urls = []
counter = 0
for i,j in zip(compnames,compadds):
g = pygoogle(i+' contact '+j)
g.pages = 1
urls.append(g.get_urls())
if (counter % 10 == 0):
with open(urlpklfile,'wb') as output:
pickle.dump(urls, output, pickle.HIGHEST_PROTOCOL)
counter += 1
sleep(np.random.uniform(5,10))
with open(urlpklfile,'wb') as output:
pickle.dump(urls, output, pickle.HIGHEST_PROTOCOL)
os.chdir(rootdir)
#urls = [[u'https://www.facebook.com/andrea.shaw.564', u'https://www.facebook.com/dianne.schultz1', u'http://www.192.com/atoz/business/brentwood/financial--advisers--(independent)/', u'https://classictvhistory.wordpress.com/tag/have-gun-will-travel/', u'http://i.dujour.com/december-print/', u'http://www.greenvillecountybar.org/Gbar_News_PDF/2014/122014.pdf', u'http://dartmouthalumnimagazine.com/class-notes/1970/all', u'http://www.dls.org/pdf/magazine/october_2007_magazine.pdf'], [u'http://www.city-data.com/clackamas-county/D/Delenka-Lane-2.html', u'http://law.justia.com/cases/alaska/supreme-court/2011/', u'https://www.facebook.com/htmody', u'https://www.facebook.com/terry.meyers.5', u'http://www.ciwf.com/media/1141326/outofsight-full-report.pdf', u'http://www.losfoundation.org/wp-content/uploads/2013/06/Donors-2011_2012.pdf', u'http://svcf.org/help/recognition/', u'https://www.ipo.gov.uk/t-tmj/tm-journals/2015-007/owner.html'], [u'https://www.sc.com/uk/contact-us/', u'https://www.sc.com/en/contact-us/', u'https://www.sc.com/je/contact-us/index.html', u'https://www.sc.com/hk/investor-relations/_documents/en/news/20130905d.pdf', u'http://www.aim25.ac.uk/cgi-bin/vcdf/detail?coll_id=18442&inst_id=118&nv1=search&nv2=', u'http://www.bloomberg.com/research/stocks/people/person.asp?personId=8307423&ticker=STAN:LN', u'http://www.hkexnews.hk/listedco/listconews/sehk/2015/0519/LTN20150519338.pdf', u'http://www.sebi.gov.in/dp/stdchtdrhp.pdf'], [u'https://www.facebook.com/theoldglovefactorymarketplace', u'https://www.grinnell.edu/about/visit/spaces/old-glove-factory', u'http://en.wikipedia.org/wiki/GlaxoSmithKline', u'http://www.dailykos.com/story/2013/01/06/1163848/-KosAbility-Trying-to-Clean-Out-an-Old-House-with-Arthritis-and-Asthma', u'http://www.cdc.gov/NCEH/publications/books/housing/cha05.htm', u'http://www.slideshare.net/MedlineIndustriesInc/surgical-gloves-a-comprehensive-guide', u'http://www.cpsc.gov/pagefiles/112284/5015.pdf', u'http://ftp.asahq.org/publicationsAndServices/latexallergy.pdf'], [u'http://www.thegsa.co.za/index.php?nav=destination_country&view=28', u'https://www.facebook.com/anna.brass1'], [], [u'http://books.openedition.org/obp/326', u'http://www.hrblock.com/tax-offices/local-offices/#!/en/office-profile/12546', u'http://www.caicv.org/dev/data/fckeditor/cms/file/Quorum_July2010WEB.pdf', u'https://play.google.com/store/apps/details?id=com.mhriley.spendingtracker&hl=en', u'https://www.facebook.com/walter.kajer.1', u'http://duchyofcornwall.org/assets/images/documents/Poundbury_Factsheet_2013.pdf', u'http://www.lihp.org/Content/2011 annual report.pdf', u'http://www.kildare.ie/business/directory/list-companies.asp?Category=Business Services'], [u'http://cera.govt.nz/sites/default/files/common/tc3-residential-rebuild-booklet-A4-20121204.pdf', u'http://www.thomsonlocal.com/Funeral-Directors/in/Surrey/', u'http://www.britishculinaryfederation.co.uk/bcf/wp-content/uploads/2011/06/091124_Culinary_News_December_v6.pdf', u'http://www.hackney.gov.uk/Assets/Documents/ht276.pdf', u'http://www.insightpublications.com.au/pdf_preview/isp-julius-caesar-10-pages.pdf', u'http://www.tripadvisor.co.uk/Hotel_Review-g191252-d491974-Reviews-Trimstone_Manor_Country_House_Hotel-Ilfracombe_Devon_England.html', u'http://www.lincoln.ac.nz/Documents/LEaP/WMK ICRF Final May 2013.pdf', u'http://delvinvillage.com/directory/'], [u'http://www.deloitte.com/', u'http://www.schencksc.com/2015rpctour/', u'http://www.schencksc.com/2013recforum/', u'https://www.linkedin.com/in/jeffreyshlefstein', u'http://www.aicpa.org/BecomeACPA/Pages/InternshipsandCooperativePrograms.aspx', u'http://www.freshbooks.com/accountants/map', u'http://www.mncpa.org/find-a-cpa/cpa-yellow-pages/list.aspx?l=c', u'http://cdn.colorado.gov/cs/Satellite?blobcol=urldata&blobheadername1=Content-Disposition&blobheadername2=Content-Type&blobheadervalue1=inline;+filename="March+28,+2007+Board+Meeting+Minutes.pdf"&blobheadervalue2=application/pdf&blobkey=id&blobtable=MungoBlobs&blobwhere=1251832310203&ssbinary=true'], []]
#urls =[[u'http://www.192.com/atoz/business/brentwood/financial--advisers--(independent)/', u'http://www.ucl.ac.uk/consultants/homepage'], [u'http://www.contactps.ca/', u'https://411.ca/business/profile/7759616'], [u'https://www.sc.com/en/contact-us/', u'https://www.sc.com/', u'https://www.sc.com/je/contact-us/index.html', u'https://www.sc.com/hk/investor-relations/_documents/en/news/20090902a.pdf', u'http://www.sebi.gov.in/dp/stdchtdrhp.pdf', u'http://www.bloomberg.com/research/stocks/people/person.asp?personId=8307423&ticker=STAN:LN', u'http://vpr.hkma.gov.hk/pdf/100269/fd_int/fd_int_0613_pt01.pdf', u'http://www.fogl.com/fogl/uploads/companypresentations/annual_report_2012.pdf'], [], [u'https://openaccess.adb.org/bitstream/handle/11540/1651/Volume 28_No 2_2011_06.pdf?sequence=1', u'http://yourtireshopsupply.com/manufacturer/27/grey-pneumatic-corp', u'https://www.facebook.com/people/\xe0\xb8\xa8\xe0\xb8\xb4\xe0\xb8\xa3\xe0\xb8\xb4\xe0\xb8\xa3\xe0\xb8\xb1\xe0\xb8\x95\xe0\xb8\x99\xe0\xb9\x8c-\xe0\xb8\x97\xe0\xb8\xa7\xe0\xb8\xb4\xe0\xb8\xa7\xe0\xb8\xb1\xe0\xb8\x92\xe0\xb8\x99\xe0\xb9\x8c/100004117395751', u'https://th-th.facebook.com/donnapa.apple', u'https://www.facebook.com/sasesopit.muttamara', u'https://th-th.facebook.com/KLShopbymarie', u'https://th-th.facebook.com/soraya.lomsungnoen.1', u'https://th-th.facebook.com/namthip.bunthong.7'], [u'http://agra-alliance.org/download/53396d7f2a934/', u'https://www.africare.org/wp-content/uploads/2014/08/AFSRNo4_BrysonEley_SuccessStoryGuide_Final_Jan7_2008_updated_June08.pdf'], [u'https://www.clearbooks.co.uk/directory/business', u'https://www.tapa.co.uk/the-tapa-opt-out-ledger.php', u'http://www.dailymail.co.uk/health/article-1330839/Blundering-doctors-leave-mother-terrified-falsely-diagnosing-brain-haemorrhage.html'], [u'http://www.priorygroup.com/location-results/item/the-priory-hospital-glasgow', u'http://www.yell.com/biz/1st-choice-plumbing-and-heating-glasgow-901468909/', u'https://www2.deloitte.com/content/dam/Deloitte/global/Documents/Consumer-Business/gx-cb-global-powers-of-retailing.pdf', u'http://www.rightmove.co.uk/property-for-sale/property-30497721.html', u'http://www.hazelwood.glasgow.sch.uk/', u'https://plus.google.com/+Paranetuklimited', u'http://www.kinningparkcomplex.org/projects-overview/bike-project/', u'https://www.glasgow.gov.uk/CHttpHandler.ashx?id=14911&p=0'], [u'http://www.scleeaccountant.com/', u'http://www.192.com/places/sk/sk8-1/sk8-1nq/', u'https://www.icpas.org/hc-career-center.aspx?id=21550', u'https://www.linkedin.com/pub/leona-crouch/26/b42/b17', u'http://www.burkertvaluation.com/wp-content/uploads/2014/04/Rpb-Vitae_General.pdf', u'http://www.alec.co.uk/cvtips/examgrcv.htm', u'http://www.chaos.umd.edu/misc/origplates.html', u'http://www.atiner.gr/bio/Syrrakos.doc'], [u'https://uk.linkedin.com/pub/david-wasilewski/27/143/368']]
# TO USE A HAND-PICKED SET OF URLS TO AVOID REPEAT REQUESTS TO GOOGLE, WHICH GET YOU BLOCKED
urls = [[u'http://www.192.com/atoz/business/brentwood/financial--advisers--(independent)/'], [u'http://www.plantmethods.com/content/10/October/2014', u'http://www.plantmethods.com/content?page=2&itemsPerPage=25'], [u'https://www.sc.com/uk/contact-us/', u'https://www.sc.com/en/contact-us/', u'https://www.sc.com/je/contact-us/index.html', u'https://www.sc.com/hk/investor-relations/_documents/en/news/20130905d.pdf', u'https://www.sc.com/hk/investor-relations/_documents/en/news/20140520b.pdf', u'http://www.bloomberg.com/research/stocks/people/person.asp?personId=8307423&ticker=STAN:LN', u'http://www.sebi.gov.in/dp/stdchtdrhp.pdf', u'http://www.hkexnews.hk/listedco/listconews/sehk/2015/0519/LTN20150519338.pdf'], [u'http://www.nhs.uk/Services/Trusts/Pharmacies/DefaultView.aspx?id=89768', u'http://www.boots.com/'], [], [], [u'https://www.xero.com/', u'http://www.sage.com/'], [u'http://www.mastercard.us/', u'http://www.baxterstorey.co.uk/'], [u'http://www.192.com/places/sk/sk8-1/sk8-1nq/', u'http://www.ey.com/', u'http://www.grantthornton.com/'], []]
#print len(urls)
#pprint(urls)
#filteredurls = urls[:]
#for count,i in enumerate(filteredurls[:]):
# for j in i:
# print j
# if ('contact' not in j):
# filteredurls[count].remove(j)
# print "NOT FOUND"
#print j
#print filteredurls[count]
#print filteredurls
# This one exceeds maximum recursion
#def empty(seq):
# try:
# return all(map(empty, seq))
# except TypeError:
# return False
def empty(seq):
"""Check if a nested list (list of lists) is completely empty, if so return 'True'"""
containslist = []
for i in range(0,len(seq)-1):
if seq[i]:
containslist.append(False)
else:
containslist.append(True)
if (False in containslist):
return False
else:
return True
def filtering(initem):
""" Check if string 'contact' is in URL, if so split by it and keep first part, else return empty list"""
if ('contact' in initem):
return initem.split('contact')[0]
else:
return []
filteredurls = [np.nan]*len(urls)
for i in range(0,len(urls)-1):
filteredurls[i] = [filtering(j) for j in urls[i]]
if empty(filteredurls[i]):
#if not filteredurls[i]:
filteredurls[i] = np.nan
#pprint(filteredurls)
#filteredurls = urls[:]
#for i,j in enumerate(urls):
# toremove = [k for k in urls[i] if 'contact' not in urls[i]]
# for l in j:
# if(j in toremove):
# filteredurls[i].remove(j)
#print filteredurls
d = {'CompanyName' : pan.Series(compnames), 'CompanyAddress1' : pan.Series(compadds), 'URLs' : pan.Series(filteredurls)}
dfurls = pan.DataFrame(d)
dfurls
#urls = [pygoogle(i).get_urls()[0] for i in list(smalldata['CompanyName'].values)]
#print urls
#smalldata['WebURL'] = Series([pygoogle(i).get_urls()[0] for i in data['CompanyName']], index=smalldata.index)
#compnames = smalldata.iterrows()[1]
#print compnames
#for i in range(0,len(smalldata)-1):
import re
from mechanize import Browser
# http://stackoverflow.com/questions/1011975/how-to-get-links-on-a-webpage-using-mechanize-and-open-those-links
def findAboutUs(inputlink):
"""Given an initial (hopefully, homepage) URL, look for an 'About Us' link, if not found just return initial URL."""
if (inputlink == np.nan):
return np.nan
#print inputlink
br = Browser()
br.open(inputlink)
aboutuslinks = []
# br.links(url_regex="about")
# br.links(text_regex="About( us)?")
for link in br.links(text_regex="About"):
#print inputlink, link.url
aboutuslinks.append(link)
#br.follow_link(link) # takes EITHER Link instance OR keyword args
#br.back()
#print aboutuslinks
# http://stackoverflow.com/questions/10994251/mechanize-urllib-beautifulsoup-relative-paths
for i,j in enumerate(aboutuslinks):
"""Mechanize often returns relative links, split into .base_url and .url We join them -if necessary- here."""
domain = re.search('(http:\/\/.*\.\D+?|https:\/\/.*\.\D+?)\/',j.base_url.strip())
if domain:
domain = domain.group(1)
if re.search('mailto',j.url.strip()) != None:
pass
elif re.search('(http:\/\/.*\.\D+?|https:\/\/.*\.\D+?)\/',j.url.strip()) != None:
u = j.url.strip()#.encode('utf8')
elif re.search('^/',j.url.strip()) != None:
u = domain+j.url.strip()#.encode('utf8')
else:
u = domain+'/'+j.url.strip()#.encode('utf8')
aboutuslinks[i] = u
# Some non-About Us links somehow still make it here, filter them out by requiring an 'about' in the URL
#print aboutuslinks
aboutuslinks = [i for i in aboutuslinks if 'about' in i]
#print aboutuslinks
# If multiple 'About Us' links found (sometimes duplicates), take the first one only
if (aboutuslinks and isinstance(aboutuslinks, list)):
aboutuslink = aboutuslinks[0]
else:
aboutuslink = aboutuslinks
# If no 'About us' link is found return initial (input) link
if aboutuslink:
return aboutuslink
else:
return inputlink
#print findAboutUs("https://www.sc.com/uk/")
print findAboutUs("http://www.growthintel.com")
#from lxml import html
#import requests
#page = requests.get('https://www.sc.com/uk/')
#tree = html.fromstring(page.text)
#print tree
#from BeautifulSoup import BeautifulSoup
#import bs4
from bs4 import BeautifulSoup
import urllib
def retrieveText(inputlink):
"""Fetch the text from a link to an HTML file"""
if (inputlink == np.nan):
return np.nan
html = urllib.urlopen(inputlink).read()
soup = BeautifulSoup(html)
texts = soup.findAll(text=True)
# http://stackoverflow.com/questions/1936466/beautifulsoup-grab-visible-webpage-text
#def visible(element):
# if element.parent.name in ['style', 'script', '[document]', 'head', 'title']:
# return False
# elif element.parent.name isinstance(element, Comment):
# #elif re.match('<!--.*-->', str(element)):
# return False
# return True
#visible_texts = filter(visible, texts)
[s.extract() for s in soup(['style', 'script', '[document]', 'head', 'title'])]
visible_text = soup.getText()
return visible_text
#print retrieveText('https://www.sc.com/uk/about-us/index.html')
print retrieveText('http://www.growthintel.com/about-us/')
def createDescription(inputlink):
"""Link the findAboutUs() and retrieveText() functions to obtain company description from input link"""
if (isinstance(inputlink,list)):
inputlink = inputlink[0]
if (inputlink == np.nan):
return np.nan
else:
link = findAboutUs(inputlink)
text = retrieveText(link)
return text
#if (isinstance(inputlinks,list)):
# link = findAboutUs("http://portent.io")
# link = findAboutUs(inputlinks[0])
#else:
# link = findAboutUs(inputlinks)
#text = retrieveText(link)
#return text
#print createDescription(np.nan)
#print createDescription("https://www.sc.com/uk/")
testlink = "http://www.growthintel.com"
print createDescription(testlink)
##dfurls = dfurls.drop('CompanyDescription', 1)
#print dfurls[ pan.notnull(dfurls['URLs']) ]
#dfurls['AboutUsURL'] = dfurls['URLs'].apply(lambda x: findAboutUs(x))
#dfurls = dfurls.drop('AboutUsURL', 1)
#dfurls['CompanyDescription'] = dfurls['URLs'].apply(lambda x: createDescription(x))
#dfurls
#print dfurls.ix[dfurls['CompanyName'] == 'STANDARD CHARTERED NOMINEES LIMITED', 'CompanyDescription'].values
#os.chdir(datadir)
#descpklfile="descriptions.pkl"
#descfolderpath=os.path.join(datadir,descpklfile)
#if (os.path.exists(descfolderpath)==True):
# print("Pickle file containing company descriptions data found. Loading it...")
# dfurls=pickle.load(open(descfolderpath,'r'))
#else:
# print("Fetching company descriptions...")
# dfurls['CompanyDescription'] = dfurls['URLs'].apply(lambda x: createDescription(x))
# with open(descpklfile,'wb') as output:
# pickle.dump(dfurls, output, pickle.HIGHEST_PROTOCOL)
#os.chdir(rootdir)
#dfurls
AboutUsURLs = [["McKinsey & Company", "http://www.mckinsey.com/about_us"], ["The White Company", "http://www.thewhitecompany.com/help/our-story/"], ["Marks & Spencer", "http://corporate.marksandspencer.com/aboutus"], ["Kids Company", "http://www.kidsco.org.uk/about-us"], ["Thunderhead", "http://www.thunderhead.com/what-we-do/about-us/"], ["Aston Martin", "https://www.astonmartin.com/en/company/about-us"], ["Bicester Village", "http://www.bicestervillage.com/en/company/about-us"], ["Solarcentury", "http://www.solarcentury.com/uk/about-solarcentury/"], ["Student Loans Company", "http://www.slc.co.uk/about-us.aspx"], ["The Stationers' Company", "https://stationers.org/about.html"], ["Royal Shakespeare Company", "http://www.rsc.org.uk/about-us/"], ["Snell", "http://www.snellgroup.com/company/about-us/"], ["The Wax Chandlers Company", "http://www.waxchandlers.org.uk/about-us/index.php"], ["Expeditors", "http://www.expeditors.com/our-company/about-us.asp"], ["The Carbon Neutral Company", "http://www.carbonneutral.com/about-us"], ["The Pewterers' Company", "http://www.pewterers.org.uk/the_company/aboutus.html"], ["Vauxhall", "http://www.vauxhall.co.uk/about-vauxhall/about-us/company.html"], ["EE", "http://ee.co.uk/our-company/about-ee"], ["Candoco Dance Company", "http://www.candoco.co.uk/about-us/"], ["Victrex", "http://www.victrex.com/en/company/about-us"], ["Ensus", "http://www.ensus.co.uk/Company/About_us/"], ["Anglian Water", "http://www.anglianwater.co.uk/about-us/"], ["The Cheque and Credit Clearing Company", "http://www.chequeandcredit.co.uk/about_us/"], ["Vodafone", "http://www.vodafone.co.uk/about-us/company-history/"], ["People 1st","http://www.people1sttraining.co.uk/about-us"], ["Starbucks","http://www.starbucks.co.uk/about-us"], ["Merlin Entertainments","http://www.merlinentertainments.biz/about-us"], ["Bloomsbury Publishing","http://www.bloomsbury.com/uk/company/about-us/"], ["Alcatel One Touch","http://www.alcatelonetouch.com/global-en/company/aboutus.html"], ["Masons Kings","http://masonkings.jd-dealer.co.uk/About-us/Our-Company"], ["Oxford Bus Company","http://www.oxfordbus.co.uk/about-us/"], ["Patient.co.uk","http://www.patient.co.uk/about-us"], ["Bootstrap Company","http://www.bootstrapcompany.co.uk/about-us/"], ["Fusion Furniture","http://www.fusionfurniturecompany.co.uk/about.php"], ["Siemens","http://www.siemens.co.uk/en/about_us/"], ["Bosch UK","http://www.bosch.co.uk/en/uk/about_bosch_home_2/about-bosch-in-great-britain.php#"], ["Qualcomm","https://www.qualcomm.com/company/about"], ["Apple","https://www.apple.com/about/"], ["Mercedes-Benz UK","http://www2.mercedes-benz.co.uk/content/unitedkingdom/mpc/mpc_unitedkingdom_website/en/home_mpc/passengercars/home/passenger_cars_world/about_us.html"], ["IBM UK","http://www.ibm.com/ibm/uk/en/"], ["Google","https://www.google.co.uk/about/"], ["Intel","http://www.intel.com/content/www/us/en/company-overview/company-overview.html"], ["ebay","http://pages.ebay.co.uk/aboutebay.html"], ["WebMD","http://www.webmd.com/about-webmd-policies/about-who-we-are"], ["Growth Intelligence","http://www.growthintel.com/about-us/"] ]
#pprint(AboutUsURLs)
print len(AboutUsURLs)
cnames = [i for i,j in AboutUsURLs]
caboutusurls = [j for i,j in AboutUsURLs]
#print cnames
descdict = {'CompanyName' : pan.Series(cnames), 'AboutUsURL' : pan.Series(caboutusurls)}
descdf = pan.DataFrame(descdict)
descdf
os.chdir(datadir)
descpklfile="descriptions.pkl"
descfolderpath=os.path.join(datadir,descpklfile)
if (os.path.exists(descfolderpath)==True):
print("Pickle file containing company descriptions data found. Loading it...")
descdf=pickle.load(open(descfolderpath,'r'))
else:
print("Fetching company descriptions...")
descdf['CompanyDescription'] = descdf['AboutUsURL'].apply(lambda x: retrieveText(x))
with open(descpklfile,'wb') as output:
pickle.dump(descdf, output, pickle.HIGHEST_PROTOCOL)
os.chdir(rootdir)
descdf
descdf
#print descdf.ix[descdf['CompanyName'] == 'Starbucks', 'CompanyDescription'].values
print descdf.ix[descdf['CompanyName'] == 'Starbucks', 'CompanyDescription'].values[0].encode('utf-8')
from nltk.corpus import stopwords
from nltk.tokenize import WordPunctTokenizer
from nltk.tokenize import PunktWordTokenizer
#from nltk.tokenize import RegexpTokenizer
from nltk.stem.snowball import EnglishStemmer
from nltk.stem.snowball import PorterStemmer
from nltk.stem.lancaster import LancasterStemmer
from nltk.stem import WordNetLemmatizer
english_stops = set(stopwords.words('english'))
def tokenizeString(string,lower=True,tokenizer="wordpunct"):
if tokenizer=="wordpunct":
tokenized=WordPunctTokenizer().tokenize(string)
if lower==True:
tokenized=[w.lower() for w in tokenized]
if tokenizer=="punktword":
tokenized=PunktWordTokenizer().tokenize(string)
if lower==True:
tokenized=[w.lower() for w in tokenized]
return tokenized
def cleanVector(tokens,clean=True,stopremove=True,minlen=2):
output=[]
disallowedchar=set(["!","?",'"',"'",",",".",":",";"])
english_stops = set(stopwords.words('english'))
for i in tokens:
found=False
if len(set(i).intersection(disallowedchar))>0:
found=True
if found==False and stopremove==False:
output.append(i)
if found==False and stopremove==True and minlen==0:
if i not in english_stops:
output.append(i)
if found==False and stopremove==True and minlen>0:
if i not in english_stops and len(i)>=minlen:
output.append(i)
return output
def stemVector(vector,method="lemmatize"):
output=[]
if method=='lemmatize':
wnl = WordNetLemmatizer()
for i in vector:
i=wnl.lemmatize(i)
output.append(i)
if method=='snowball':
st=EnglishStemmer()
for i in vector:
i=st.stem(i)
output.append(i)
if method=='porter':
st=PorterStemmer()
for i in vector:
i=st.stem(i)
output.append(i)
if method=='lancaster':
st=LancasterStemmer()
for i in vector:
i=st.stem(i)
output.append(i)
return output
def tokeniseCleanStem(inputstring):
return stemVector(cleanVector(tokenizeString(inputstring)))
os.chdir(datadir)
descpklfile="processeddescriptions.pkl"
descfolderpath=os.path.join(datadir,descpklfile)
if (os.path.exists(descfolderpath)==True):
print("Pickle file containing preprocessed company data found. Loading it...")
descdf=pickle.load(open(descfolderpath,'r'))
else:
print("Cleaning, tokenising and lemmatising company data text...")
descdf['Tokens'] = descdf['CompanyDescription'].apply(lambda x: tokeniseCleanStem(x))
with open(descpklfile,'wb') as output:
pickle.dump(descdf, output, pickle.HIGHEST_PROTOCOL)
os.chdir(rootdir)
descdf
#print descdf['Tokens']
from gensim import corpora,models
dictionary = corpora.Dictionary(descdf['Tokens'])
print dictionary
#print(dictionary.token2id)
corpus = [dictionary.doc2bow(text) for text in descdf['Tokens']]
#print(corpus)
tfidfmodel = models.TfidfModel(corpus)
# Apply it to the input corpus
tfidfcorpus = tfidfmodel[corpus]
#print(tfidfcorpus)
dictpath = os.path.join(datadir,'companies.dict')
dictionary.save(dictpath)
corpuspath = os.path.join(datadir,'corpus.mm')
corpora.MmCorpus.serialize(corpuspath, corpus)
import logging
logging.basicConfig(filename='companies.log', format='%(asctime)s : %(levelname)s : %(message)s', level=logging.INFO)
#id2word = corpora.Dictionary.load_from_text(dictpath)
id2word = dictionary
#mm = corpora.MmCorpus(corpuspath)
mm = tfidfcorpus
lda = models.ldamodel.LdaModel(corpus=mm, id2word=id2word, num_topics=2, update_every=1, chunksize=10000, passes=10)
ldapath = os.path.join(datadir,'companies_lda.model')
lda.save(ldapath)
lda.print_topics(10)
from gensim.similarities import Similarity
from gensim import similarities
query = "Electronics appliances"
query = dictionary.doc2bow(tokeniseCleanStem(query))
# Apply the LDA model trained on the corpus to the query
query_lda = lda[query]
print "\nThe similarity of the query with each one of the computed topics is:\n"
print(query_lda)
index = similarities.MatrixSimilarity(lda[tfidfcorpus])
print "\n\nThe similarity of the query to the documents in the corpus is:\n"
sims = index[query_lda] # perform a similarity query against the corpus
resultlist = list(enumerate(sims))
print(resultlist)
print "\n\nThe company which best fits the query by LDA-deduced topics is:\n"
resultlist.sort(key=lambda x: x[1], reverse=True)
result = resultlist[0][0]
print descdf.iloc[result]
###Output
WARNING:gensim.similarities.docsim:scanning corpus to determine the number of features (consider setting `num_features` explicitly)
|
Probability & Statistics/Pratical Statistics/01.Stimulating Coin Flips - Probability.ipynb | ###Markdown
Stimulating Coin Flips
###Code
import numpy as np
np.random.rand()
###Output
_____no_output_____
###Markdown
Flipping coins x times
###Code
#100 times coin flip
np.random.randint(5, size = 100)
###Output
_____no_output_____
###Markdown
Flipping Weighted Coins
###Code
coin_faces = [0, 1]
# heads for 80%, tail for 20%
np.random.choice(coin_faces, size = 100, p = [0.8, 0.2])
np.random.choice(coin_faces, size = 100, p = [0.8, 0.2]).mean()
###Output
_____no_output_____
###Markdown
---------- More Exercises
###Code
# outcome of one coin flip
np.random.randint(2)
# outcomes of ten thousand coin flips
np.random.randint(2, size = 10000)
# mean outcome of ten thousand coin flips
np.random.randint(2, size = 10000).mean()
###Output
_____no_output_____
###Markdown
using choice method for Biased Coin
###Code
# outcome of one coin flip, using choice
np.random.choice([0,1])
# outcome of ten thousand coin flips
np.random.choice([0,1], size = 10000)
# mean outcome of ten thousand coin flips
np.random.choice([0,1], size = 10000).mean()
# outcomes of ten thousand biased coin flips
np.random.choice([0,1], size = 10000, p = [0.8, 0.2])
# mean outcome of ten thousand biased coin flips
np.random.choice([0, 1], size = 10000, p =[0.8, 0.2]).mean()
###Output
_____no_output_____
###Markdown
------------- Probability Quiz Coin Flips and Die Rolls0 represents heads. 1 represents tails.
###Code
import numpy as np
###Output
_____no_output_____
###Markdown
1.Two fair coin flips produce exactly two heads
###Code
# simulate 1 million tests of two fair coin flips
results = np.random.randint(2, size =(1000000, 2))
# sums of all tests
total_sum = results.sum(axis = 1)
# proportion of tests that produced exactly two heads
(total_sum == 0).mean()
###Output
_____no_output_____
###Markdown
2. Three fair coin flips produce exactly one head
###Code
# simulate 1 million tests of three fair coin flips
results = np.random.randint(2, size = (1000000, 3))
# sums of all tests
total_sum = results.sum(axis = 1)
# proportion of tests that produced exactly one head
(total_sum == 2).mean()
###Output
_____no_output_____
###Markdown
3. Three biased coin flips with P(H) = 0.6 produce exactly one head
###Code
# simulate 1 million tests of three biased coin flips
# hint: use np.random.choice()
results = np.random.choice([0,1] , size=(1000000, 3), p = [0.6, 0.4])
# sums of all tests
total_sum = results.sum(axis = 1)
# proportion of tests that produced exactly one head
(total_sum == 2).mean()
###Output
_____no_output_____
###Markdown
4. A die rolls an even number
###Code
# simulate 1 million tests of one die roll
results = np.random.choice(np.arange(1,7), size = 1000000)
results
# proportion of tests that produced an even number
# len([i for i in tests if i%2 == 0])/int(1e6)
(results % 2 == 0).mean()
len([i for i in results if i%2 == 0])/int(1e6)
###Output
_____no_output_____
###Markdown
5. Two dice roll a double
###Code
# simulate the first million die rolls
results_one = np.random.choice(np.arange(1, 7), size = int(1e6))
# simulate the second million die rolls
results_two = np.random.choice(np.arange(1, 7), size = int(1e6))
# proportion of tests where the 1st and 2nd die rolled the same number
# len([(i, j) for (i,j) in zip(first, second) if i == j]) / int(1e6)
(results_one == results_two).mean()
###Output
_____no_output_____ |
examples/ch09/snippets_ipynb/09_05.ipynb | ###Markdown
9.5 Serialization with JSON JSON Data Format Python Standard Library Module `json`
###Code
accounts_dict = {'accounts': [
{'account': 100, 'name': 'Jones', 'balance': 24.98},
{'account': 200, 'name': 'Doe', 'balance': 345.67}]}
###Output
_____no_output_____
###Markdown
Serializing an Object to JSON
###Code
import json
with open('accounts.json', 'w') as accounts:
json.dump(accounts_dict, accounts)
###Output
_____no_output_____
###Markdown
Deserializing the JSON Text
###Code
with open('accounts.json', 'r') as accounts:
accounts_json = json.load(accounts)
accounts_json
accounts_json['accounts']
accounts_json['accounts'][0]
accounts_json['accounts'][1]
###Output
_____no_output_____
###Markdown
Displaying the JSON Text
###Code
with open('accounts.json', 'r') as accounts:
print(json.dumps(json.load(accounts), indent=4))
##########################################################################
# (C) Copyright 2019 by Deitel & Associates, Inc. and #
# Pearson Education, Inc. All Rights Reserved. #
# #
# DISCLAIMER: The authors and publisher of this book have used their #
# best efforts in preparing the book. These efforts include the #
# development, research, and testing of the theories and programs #
# to determine their effectiveness. The authors and publisher make #
# no warranty of any kind, expressed or implied, with regard to these #
# programs or to the documentation contained in these books. The authors #
# and publisher shall not be liable in any event for incidental or #
# consequential damages in connection with, or arising out of, the #
# furnishing, performance, or use of these programs. #
##########################################################################
###Output
_____no_output_____ |
SHARPENING (HIGHPASS) SPATIAL FILTERS.ipynb | ###Markdown
SHARPENING (HIGHPASS) SPATIAL FILTERS
###Code
import cv2
import matplotlib.pyplot as plt
import numpy as np
###Output
_____no_output_____
###Markdown
上一部分介绍的blur能够将图片模糊化, 这部分介绍的是突出图片的边缘的细节.什么是边缘呢? 往往是像素点跳跃特别大的点, 这部分和梯度的概念是类似的, 可以如下定义图片的一阶导数而二阶导数:$$\frac{\partial f}{\partial x} = f(x+1) - f(x), \\\frac{\partial^2 f}{\partial x^2} = f(x+1) + f(x-1) - 2f(x).$$注: 或许用差分来表述更为贴切.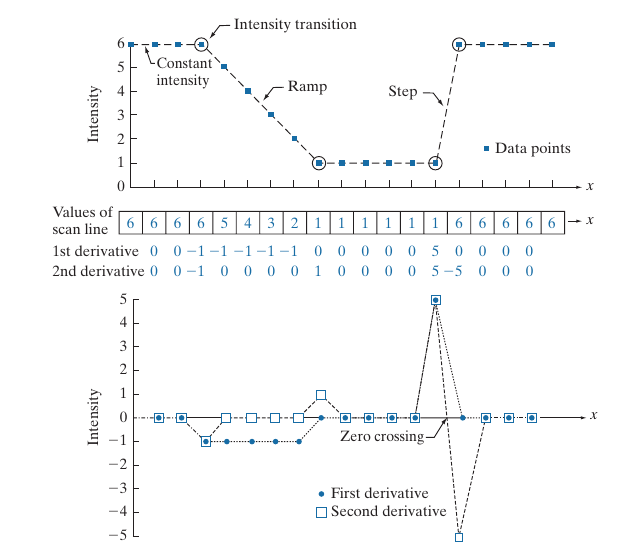如上图实例所示, 描述了密度值沿着$x$的变化, 一阶导数似乎能划分区域, 而二阶导数能够更好的“识别"边缘. Laplacian著名的laplacian算子:$$\Delta f = \frac{\partial^2 f}{\partial x^2} + \frac{\partial^2 f}{\partial y^2},$$在digital image这里:$$\Delta f = f(x+1, y) + f(x-1, y) + f(x, y+1) + f(x, y-1) - 4 f(x, y).$$这个算子用kernel表示是下面的(a), 但是在实际中也有(b, c, d)的用法, (b, d)额外用到了对角的信息, 注意到这些kernels都满足$$\sum_{ij}w_{ij} = 0.$$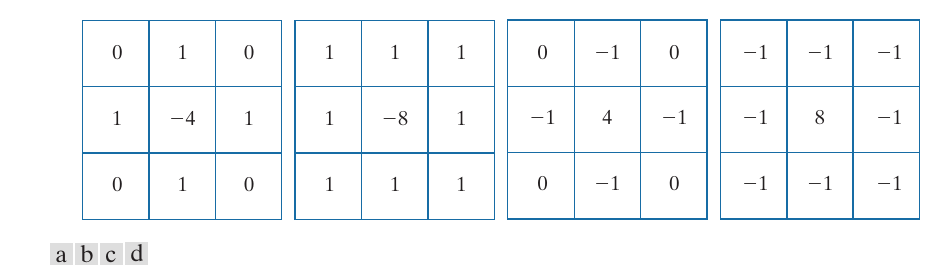
###Code
img = cv2.imread("./pics/moon.png")
img.shape
img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) # 由于是截图, 先转成灰度图
img = img.astype(np.float32)
plt.imshow(img, cmap='gray')
kernel = -np.ones((3, 3))
kernel[1, 1] = 8
laps = cv2.filter2D(img, -1, kernel)
laps = (laps - laps.min()) / (laps.max() - laps.min()) * 255
img_pos = img + laps
img_neg = img - laps
fig, axes = plt.subplots(1, 4)
axes[0].imshow(img, cmap='gray')
axes[1].imshow(laps, cmap='gray')
axes[2].imshow(img_pos, cmap='gray')
axes[3].imshow(img_neg, cmap='gray')
plt.tight_layout()
plt.show()
kernel = np.ones((3, 3))
kernel[0, 0] = 0
kernel[0, 2] = 0
kernel[1, 1] = -4
kernel[2, 0] = 0
kernel[2, 2] = 0
laps = cv2.filter2D(img, -1, kernel)
laps = (laps - laps.min()) / (laps.max() - laps.min()) * 255
img_pos = img + laps
img_neg = img - laps
fig, axes = plt.subplots(1, 4)
axes[0].imshow(img, cmap='gray')
axes[1].imshow(laps, cmap='gray')
axes[2].imshow(img_pos, cmap='gray')
axes[3].imshow(img_neg, cmap='gray')
plt.tight_layout()
plt.show()
###Output
_____no_output_____
###Markdown
UNSHARP MASKING AND HIGHBOOST FILTERING注意到, 之前的box kernel,$$w_{box}(s, t) = \frac{1}{mn},$$考虑$3 \times 3$的kernel size下:$$w_{lap} = 9(E - \cdot w_{box}),$$这里$$E(s, t) =0, \forall s\not=2, t\not=2.$$故假设$$g_{mask} (x, y) = f(x, y) - \bar{f} (x, y),$$其中$\bar{f}$是通过box filter 模糊的图像, 则$$\Delta f = 9 \cdot g_{mask}.$$故$g_{mask}$也反应了细节边缘信息.进一步定义$$g(x, y) = f(x, y) + k g_{mask}(x, y).$$
###Code
kernel = np.ones((3, 3)) / 9
img_mask = (img - cv2.filter2D(img, -1, kernel)) * 9
img_mask = (img_mask - img_mask.mean()) / (img_mask.max() - img_mask.min())
fig, ax = plt.subplots(1, 1)
ax.imshow(img_mask, cmap='gray')
plt.show()
###Output
_____no_output_____
###Markdown
First-Order Derivatives最后再说说如何用一阶导数提取细节.定义$$M(x, y) = \|\nabla f\| = \sqrt{(\frac{\partial f}{\partial x})^2 + (\frac{\partial f}{\partial y})^2}.$$注: 也常常用$M(x, y) = |\frac{\partial f}{\partial x}| + |\frac{\partial f}{\partial y}|$代替.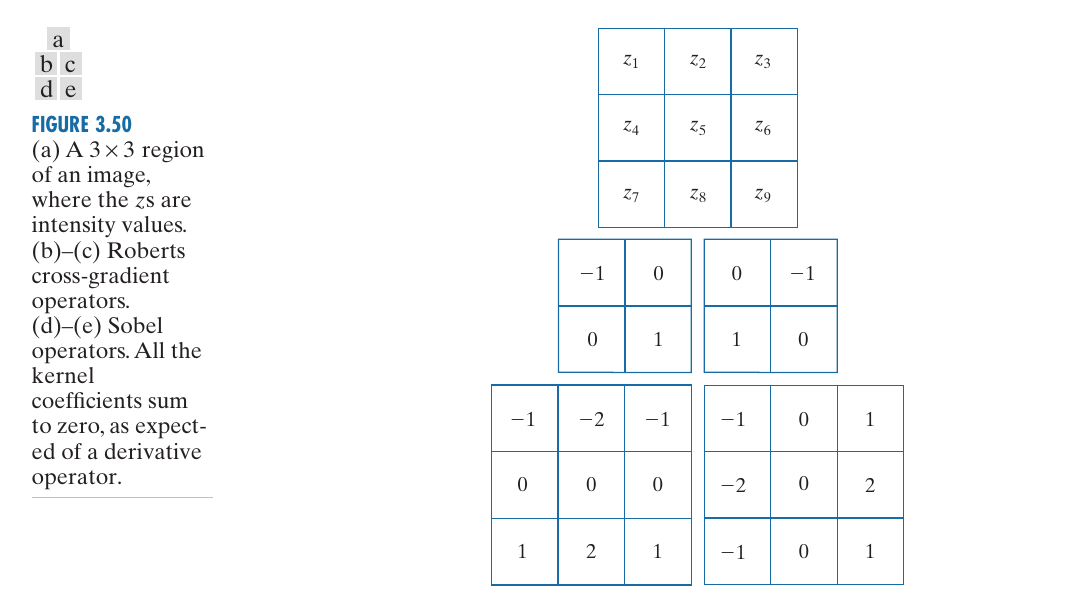 Roberts cross-gradient把目标区域按照图(a)区分, Roberts cross-gradient采用如下方式定义:$$\frac{\partial f}{\partial x} = z_9 - z_5, \: \frac{\partial f}{\partial y} = z_8 - z_6,$$即右下角的对角之差. 所以相应的kernel变如图(b, c)所示(其余部分为0, $3 \times 3$).注: 计算$M$需要两个kernel做两次卷积. Sobel operatorsSobel operators 则是$$\frac{\partial f}{\partial x} = (z_7 + 2z_8 + z_9) - (z_1 + 2z_2 + z_3) \\\frac{\partial f}{\partial y} = (z_3 + 2z_6 + z_9) - (z_1 + 2z_4 + z_7),$$即如图(d, e)所示.
###Code
kernel = np.zeros((3, 3))
kernel[1, 1] = -1
kernel[2, 2] = 1
part1 = cv2.filter2D(img, -1, kernel)
kernel = np.zeros((3, 3))
kernel[1, 2] = -1
kernel[2, 1] = 1
part2 = cv2.filter2D(img, -1, kernel)
img_roberts = np.sqrt(part1 ** 2 + part2 ** 2)
part1 = cv2.Sobel(img, -1, dx=1, dy=0, ksize=3)
part2 = cv2.Sobel(img, -1, dx=0, dy=1, ksize=3)
img_sobel = np.sqrt(part1 ** 2 + part2 ** 2)
fig, axes = plt.subplots(1, 2)
axes[0].imshow(img_roberts, cmap='gray')
axes[1].imshow(img_sobel, cmap='gray')
###Output
_____no_output_____ |
results_for_paper_fpcross/paper_plots.ipynb | ###Markdown
Constants
###Code
FILE_DATA = './fpcross_results'
FOLD_IMAG = './plot/res_'
TYPE_IMAG = 'pgf'
SAVE_IMAG = True # !!!
###Output
_____no_output_____
###Markdown
Helpers
###Code
def load(name):
with open(FILE_DATA, 'rb') as f:
res = pickle.load(f)
return res[name]
def show_prep(ax, with_leg=True, is_log=True):
if with_leg:
ax.legend(loc='best', frameon=True)
if is_log:
ax.semilogy()
ax.grid(ls=":")
ax.spines['top'].set_visible(False)
ax.spines['right'].set_visible(False)
ax.get_xaxis().tick_bottom()
ax.get_yaxis().tick_left()
def show(res):
t = [i*res['h'] for i in range(1, res['m'] + 1)]
if res['np']:
fig, ax1 = plt.subplots(nrows=1, ncols=1, figsize=(8, 8))
else:
fig, (ax1, ax2) = plt.subplots(nrows=1, ncols=2, figsize=(16, 8))
plt.subplots_adjust(wspace=0.2)
ax1.set_title('Relative error')
ax1.set_xlabel('Time')
if len(res['e_stat']):
ax1.plot(t, res['e_stat'], label='vs stationary',
linestyle='-', linewidth=2, color='#8b1d1d',
marker='o', markersize=7, markerfacecolor='#8b1d1d',
markeredgewidth=1, markeredgecolor='#8b1d1d')
if len(res['e_real']):
ax1.plot(t, res['e_real'], label='vs analytic',
linestyle='-.', linewidth=2, color='#5f91ac',
marker='o', markersize=0, markerfacecolor='#5f91ac',
markeredgewidth=0, markeredgecolor='#5f91ac')
show_prep(ax1)
if res['tt']:
ax2.set_title('TT-rank')
ax2.set_xlabel('Time')
ax2.plot(t, res['e_rank'],
linestyle='-', linewidth=2, color='#8b1d1d',
marker='o', markersize=7, markerfacecolor='#8b1d1d',
markeredgewidth=1, markeredgecolor='#8b1d1d')
show_prep(ax2, with_leg=False, is_log=False)
if SAVE_IMAG:
fpath = f'{FOLD_IMAG}{res["name"]}.{TYPE_IMAG}'
plt.savefig(fpath, bbox_inches='tight')
# plt.savefig(f'./res_{res["name"]}.png', bbox_inches='tight')
plt.show()
def show_dum(res):
t = [i*res['h'] for i in range(1, res['m'] + 1)]
fig, (ax1, ax2) = plt.subplots(nrows=1, ncols=2, figsize=(16, 8))
plt.subplots_adjust(wspace=0.2)
ax1.set_title('Computation results')
ax1.set_xlabel('Time')
ax1.plot(t, res['dum_p_list'], label='Value of $\psi$',
linestyle='-', linewidth=2, color='#5f91ac',
marker='o', markersize=7, markerfacecolor='#5f91ac',
markeredgewidth=1, markeredgecolor='#5f91ac')
ax1.plot(t, res['dum_e_list'], label='Value of $\eta$',
linestyle='-', linewidth=2, color='#8b1d1d',
marker='o', markersize=7, markerfacecolor='#8b1d1d',
markeredgewidth=1, markeredgecolor='#8b1d1d')
show_prep(ax1, is_log=False)
ax2.set_title('TT-rank')
ax2.set_xlabel('Time')
ax2.plot(t, res['e_rank'],
linestyle='-', linewidth=2, color='#8b1d1d',
marker='o', markersize=7, markerfacecolor='#8b1d1d',
markeredgewidth=1, markeredgecolor='#8b1d1d')
show_prep(ax2, with_leg=False, is_log=False)
if SAVE_IMAG:
fpath = f'{FOLD_IMAG}{res["name"]}.{TYPE_IMAG}'
plt.savefig(fpath, bbox_inches='tight')
# plt.savefig(f'./res_{res["name"]}.png', bbox_inches='tight')
plt.show()
###Output
_____no_output_____
###Markdown
OUP 1D
###Code
name = 'oup_1d_np'
res = load(name)
show(res)
###Output
findfont: Font family ['serif'] not found. Falling back to DejaVu Sans.
findfont: Font family ['monospace'] not found. Falling back to DejaVu Sans.
###Markdown
OUP 3D
###Code
name = 'oup_3d_tt'
res = load(name)
show(res)
###Output
_____no_output_____
###Markdown
OUP 5D
###Code
name = 'oup_5d_tt'
res = load(name)
show(res)
###Output
_____no_output_____
###Markdown
Dum 3D
###Code
name = 'dum_3d_tt'
res = load(name)
show_dum(res)
p_calc = res['dum_p_list'][-1]
p_real = 2.071143
e = np.abs((p_calc - p_real) / p_real)
print(f'{p_calc:6.4f}')
print(f'Error for psi v.s. "exact" : {e:-8.2e}')
e_calc = res['dum_e_list'][-1]
e_real = 1.0328125
e = np.abs((e_calc - e_real) / e_real)
print(f'{e_calc:6.4f}')
print(f'Error for eta v.s. "exact" : {e:-8.2e}')
###Output
1.0318
Error for eta v.s. "exact" : 9.65e-04
###Markdown
TMP
###Code
import numpy as np
from fpcross import init_jupyter, Model, Solver, SolversCheck
init_jupyter()
###Output
_____no_output_____
###Markdown
Plots for the paper
###Code
import sys
import numpy as np
import matplotlib as mpl
mpl.rc('font', **{'size' : 18})
mpl.rcParams.update({
"font.family": "normal",
"font.serif": [],
"font.sans-serif": [],
"font.monospace": [],
})
mpl.rcParams['text.usetex'] = True
import matplotlib.pyplot as plt
import matplotlib.ticker as ticker
import seaborn as sns
sns.set_context('paper', font_scale=3.0)
sns.set_style('white')
sns.mpl.rcParams['legend.frameon'] = 'False'
sys.path.extend(['./../lib', './../helpers'])
from config import config
from solver import Solver
from solvers_check import SolversCheck
pal = sns.color_palette()
data_path = './data/eq-1d_drift-linear_analyt'
figs_path = './../../paper_fpcross/text/fig_'
name1 = 'Solver (ord=1)'
name2 = 'Solver (ord=2)'
line_calc = {
'linestyle': '-',
'linewidth': 1,
'color': '#8b1d1d',
'marker': 'o',
'markersize': 7,
'markerfacecolor': '#8b1d1d',
'markeredgewidth': 1,
'markeredgecolor': '#8b1d1d',
}
line_appr = {
'linestyle': '-.',
'linewidth': 2,
'color': '#5f91ac',
'marker': 'o',
'markersize': 0,
'markerfacecolor': '#5f91ac',
'markeredgewidth': 0,
'markeredgecolor': '#5f91ac',
}
SLC = SolversCheck(data_path)
SLC.load()
M = SLC.res[name2]['M'].copy()
N = SLC.res[name2]['N'].copy()
###Output
_____no_output_____
###Markdown
Error (real) vs number of time points for the 2th order solver
###Code
n = N[-1]
x = np.array(M)
y = np.array([SLC.res[name2]['%d-%d'%(m, n)]['err'] for m in M])
# a, b = np.polyfit(1./x**2, y, 1)
a = +9.5E+0
z = a / x**2
s_appr = r'$9.5 \cdot m^{-2}$'
fig, ax = plt.subplots(nrows=1, ncols=1, figsize=(6, 6))
ax.plot(x, y, label='Solution', **line_calc)
ax.plot(x, z, label=s_appr, **line_appr)
ax.set_title('Solution error')
ax.set_xlabel('Number of time points')
ax.set_ylabel('')
ax.semilogx()
ax.semilogy()
ax.grid(ls=":")
ax.spines['top'].set_visible(False)
ax.spines['right'].set_visible(False)
ax.get_xaxis().tick_bottom()
ax.get_yaxis().tick_left()
ax.legend(loc='best', frameon=True)
plt.savefig(figs_path + 'eq-1d_drift-linear_analyt_e-vs-m.pgf', bbox_inches='tight')
###Output
_____no_output_____
###Markdown
Computation time vs number of time points for the 2th order solver
###Code
n = N[-1]
x = np.array(M)
y = np.array([SLC.res[name2]['%d-%d'%(m, n)]['t_calc'] for m in M])
fig, ax = plt.subplots(nrows=1, ncols=1, figsize=(6, 6))
ax.plot(x, y, label='Solution', **line_calc)
ax.set_title('Computation time')
ax.set_xlabel('Number of time points')
ax.set_ylabel('')
ax.semilogx()
ax.semilogy()
ax.grid(ls=":")
ax.spines['top'].set_visible(False)
ax.spines['right'].set_visible(False)
ax.get_xaxis().tick_bottom()
ax.get_yaxis().tick_left()
plt.savefig(figs_path + 'eq-1d_drift-linear_analyt_t-vs-m.pgf', bbox_inches='tight')
###Output
_____no_output_____
###Markdown
Error vs number of spatial points (2th order solver)
###Code
m = M[-1]
x = np.array(N, dtype='float')
y = np.array([SLC.res[name2]['%d-%d'%(m, n)]['err'] for n in N])
# b, a = np.polyfit(x[1:None], np.log(x[1:None]), 1, w=np.sqrt(y[1:None]))
# a = np.exp(a)
# s_appr = '%8.1e * exp[ %9.1e * n ]'%(a, b)
a = +3.5E+3
b = -5.7E-1
z = a * np.exp(b * x)
s_appr = '$3500 \cdot e^{-0.57 n}$'
fig, ax = plt.subplots(nrows=1, ncols=1, figsize=(6, 6))
ax.plot(x, y, label='Solution', **line_calc)
ax.plot(x, z, label=s_appr, **line_appr)
ax.set_title('Solution error')
ax.set_xlabel('Number of spatial points')
ax.set_ylabel('')
ax.semilogy()
ax.grid(ls=":")
ax.spines['top'].set_visible(False)
ax.spines['right'].set_visible(False)
ax.get_xaxis().tick_bottom()
ax.get_yaxis().tick_left()
ax.legend(loc='best', frameon=True)
plt.savefig(figs_path + 'eq-1d_drift-linear_analyt_e-vs-n.pgf', bbox_inches='tight')
###Output
_____no_output_____
###Markdown
Computation time vs number of spatial points for the 2th order solver
###Code
m = M[-1]
x = np.array(N)
y = np.array([SLC.res[name2]['%d-%d'%(m, n)]['t_calc'] for n in N])
fig, ax = plt.subplots(nrows=1, ncols=1, figsize=(6, 6))
ax.plot(x, y, label='Solution', **line_calc)
ax.set_ylim(5.0E+1, 1.1E+3)
ax.set_title('Computation time')
ax.set_xlabel('Number of spatial points')
ax.set_ylabel('')
ax.semilogy()
ax.grid(ls=":")
ax.spines['top'].set_visible(False)
ax.spines['right'].set_visible(False)
ax.get_xaxis().tick_bottom()
ax.get_yaxis().tick_left()
plt.savefig(figs_path + 'eq-1d_drift-linear_analyt_t-vs-n.pgf', bbox_inches='tight')
###Output
_____no_output_____
###Markdown
Solution in the central spatial point vs time
###Code
line_init = {
'linestyle': '--',
'linewidth': 3,
'color': '#48484c',
'marker': 'o',
'markersize': 0,
'markerfacecolor': '#48484c',
'markeredgewidth': 0,
'markeredgecolor': '#48484c',
}
line_calc = {
'linestyle': '-',
'linewidth': 1,
'color': '#8b1d1d',
'marker': 'o',
'markersize': 7,
'markerfacecolor': '#8b1d1d',
'markeredgewidth': 1,
'markeredgecolor': '#8b1d1d',
}
line_real = {
'linestyle': '-',
'linewidth': 3,
'color': '#485536',
'marker': 'o',
'markersize': 0,
'markerfacecolor': '#485536',
'markeredgewidth': 0,
'markeredgecolor': '#485536',
}
line_stat = {
'linestyle': '-.',
'linewidth': 2,
'color': '#5f91ac',
'marker': 'o',
'markersize': 0,
'markerfacecolor': '#5f91ac',
'markeredgewidth': 0,
'markeredgecolor': '#5f91ac',
}
line_err_real = {
'linestyle': '-',
'linewidth': 2,
'color': '#485536',
'marker': 'o',
'markersize': 6,
'markerfacecolor': '#8b1d1d',
'markeredgewidth': 1,
'markeredgecolor': '#8b1d1d',
}
line_err_stat = {
'linestyle': '-.',
'linewidth': 2,
'color': '#5f91ac',
'marker': '*',
'markersize': 6,
'markerfacecolor': '#5f91ac',
'markeredgewidth': 1,
'markeredgecolor': '#5f91ac',
}
x = np.array([0.])
i = SL._sind(x)
x = SL.X_hst[:, i].reshape(-1, 1)
t = SL.T_hst
v = np.ones(t.shape[0])
r_init = v * SL.func_r0(x)[0]
r_stat = v * SL.func_rs(x)[0]
r_real = np.array([SL.func_rt(x, t_)[0] for t_ in t])
r_calc = np.array([r[i] for r in SL.R_hst])
x = [SL.t_min] + list(t)
fig, ax = plt.subplots(nrows=1, ncols=1, figsize=(6, 6))
ax.plot(x, [r_init[0]] + list(r_init), label='Initial', **line_init)
ax.plot(x, [r_init[0]] + list(r_calc), label='Calculated', **line_calc)
ax.plot(x, [r_init[0]] + list(r_real), label='Analytic', **line_real)
ax.plot(x, [r_stat[0]] + list(r_stat), label='Stationary', **line_stat)
ax.set_title('PDF value at the point')
ax.set_xlabel('Time')
ax.semilogy()
ax.grid(ls=":")
ax.spines['top'].set_visible(False)
ax.spines['right'].set_visible(False)
ax.get_xaxis().tick_bottom()
ax.get_yaxis().tick_left()
ax.legend(loc='best', frameon=True)
plt.savefig(figs_path + 'eq-1d_drift-linear_analyt_x-zero-vs-t-res.pgf', bbox_inches='tight')
e0 = np.abs(r_stat[0] - r_init[0]) / np.abs(r_stat[0])
e_stat = [e0] + list(np.abs(r_stat - r_calc) / np.abs(r_stat))
e_real = [0.] + list(np.abs(r_real - r_calc) / np.abs(r_real))
fig, ax = plt.subplots(nrows=1, ncols=1, figsize=(6, 6))
ax.plot(x, e_real, label='vs analytic', **line_err_real)
ax.plot(x, e_stat, label='vs stationary', **line_err_stat)
ax.set_title('Relative error at the point')
ax.set_xlabel('Time')
ax.semilogy()
ax.grid(ls=":")
ax.spines['top'].set_visible(False)
ax.spines['right'].set_visible(False)
ax.get_xaxis().tick_bottom()
ax.get_yaxis().tick_left()
ax.legend(loc='best', frameon=True)
plt.savefig(figs_path + 'eq-1d_drift-linear_analyt_x-zero-vs-t-err.pgf', bbox_inches='tight')
###Output
_____no_output_____
###Markdown
Solution at the final time moment
###Code
i = -1
t = SL.T_hst[i]
x = SL.X_hst
r_init = SL.func_r0(x)
r_stat = SL.func_rs(x)
r_real = SL.func_rt(x, t)
r_calc = SL.R_hst[i]
x = x.reshape(-1)
fig, ax = plt.subplots(nrows=1, ncols=1, figsize=(6, 6))
ax.plot(x, r_init, label='Initial', **line_init)
ax.plot(x, r_calc, label='Calculated', **line_calc)
ax.plot(x, r_real, label='Analytic', **line_real)
ax.plot(x, r_stat, label='Stationary', **line_stat)
ax.set_title('PDF values at the final moment')
ax.set_xlabel('Spatial coordinate')
ax.semilogy()
ax.grid(ls=":")
ax.spines['top'].set_visible(False)
ax.spines['right'].set_visible(False)
ax.get_xaxis().tick_bottom()
ax.get_yaxis().tick_left()
ax.legend(loc='best', frameon=True)
plt.savefig(figs_path + 'eq-1d_drift-linear_analyt_t-end-vs-x-res.pgf', bbox_inches='tight')
e_real = np.abs(r_real - r_calc) / np.abs(r_real)
e_stat = np.abs(r_stat - r_calc) / np.abs(r_stat)
fig, ax = plt.subplots(nrows=1, ncols=1, figsize=(6, 6))
ax.plot(x, e_real, label='vs analytic', **line_err_real)
ax.plot(x, e_stat, label='vs stationary', **line_err_stat)
ax.set_title('Relative error at the final moment')
ax.set_xlabel('Spatial coordinate')
ax.semilogy()
ax.grid(ls=":")
ax.spines['top'].set_visible(False)
ax.spines['right'].set_visible(False)
ax.get_xaxis().tick_bottom()
ax.get_yaxis().tick_left()
ax.legend(loc='best', frameon=True)
plt.savefig(figs_path + 'eq-1d_drift-linear_analyt_t-end-vs-x-err.pgf', bbox_inches='tight')
###Output
_____no_output_____
###Markdown
Check stationary solution We calculate rhs (for the large value $\tau$ of time)$$ rhs(x) = \sum_{i=1}^d \sum_{j=1}^d \frac{\partial^2}{\partial x_i \partial x_j} \left[ D_{ij}(x, \tau) \rho_{stat}(x) \right] - \sum_{i=1}^d \frac{\partial}{\partial x_i} \left[ f_i(x, \tau) \rho_{stat}(x) \right],$$using Chebyshev grid and Chebyshev differential matrices ($D_1$, $D_2$)$$ \begin{split} rhs & = D_c (D_2 \otimes I \otimes \ldots \otimes I) \rho_{stat} + \ldots + D_c (I \otimes I \otimes \ldots \otimes D_2) \rho_{stat} - \\ & - (D_1 \otimes I \otimes \ldots \otimes I) ( f_1 \rho_{stat} ) - \ldots - (I \otimes I \otimes \ldots \otimes D_1) ( f_d \rho_{stat} ), \end{split}$$and check if it close to zero.
###Code
from intertrain import Intertrain
IT = Intertrain(n=[x_poi], l=[[x_min, x_max]], with_tt=False)
IT.init(func_rs)
I0 = np.eye(x_poi)
J0 = np.eye(x_poi); J0[0, 0] = 0.; J0[-1, -1] = 0.
D1 = IT.dif1()
D2 = IT.dif2()
t = 10.
x = IT.grid()
f = func_f0(x, t)
r = IT.Y.reshape(-1, order='F')
rhs = D_coef * D2 @ r
rhs-= D1 @ (f[0, :] * r)
print('Norm of rho : %-8.2e'%np.linalg.norm(r))
print('Norm of rhs : %-8.2e'%np.linalg.norm(rhs))
###Output
Norm of rho : 1.60e+00
Norm of rhs : 2.53e-13
|
code/phase0/3.1.Train-TF-DDP.ipynb | ###Markdown
[Module 3.1] SageMaker DDP 모델 훈련 중요 사항- 이 예시는 노트북 인스턴스가 **ml.p3.16xlarge** 에서만 동작 합니다.- 본 워크샵의 모든 노트북은 **conda_tensorflow2_p36** 를 사용합니다.이 노트북은 아래와 같은 작업을 합니다.- 1. 기본 환경 세팅 - 2. 데이터 세트를 S3 에 업로딩- 3. 노트북에서 세이지 메이커 스크립트 모드 스타일로 코드 변경- 4. 세이지 메이커 로컬 모드로 훈련- 5. 세이지 메이커의 호스트 모드로 훈련- 6. 모델 아티펙트 경로 저장 참고:- 세이지 메이커의 공식 개발자 가이드 입니다. - [개발자 가이드](https://docs.aws.amazon.com/sagemaker/latest/dg/distributed-training.html)- 세이지 메이커 분산 라이브러리 예세 Git 입니다. - [세이지 메이커 분산 라이브러리 공식 예제](https://github.com/aws/amazon-sagemaker-examples/tree/master/training/distributed_training)--- 1. 기본 세팅사용하는 패키지는 import 시점에 다시 재로딩 합니다.
###Code
%load_ext autoreload
%autoreload 2
import sagemaker
sagemaker_session = sagemaker.Session()
bucket = sagemaker_session.default_bucket()
prefix = "sagemaker/DEMO-pytorch-cnn-cifar10"
role = sagemaker.get_execution_role()
import os
import subprocess
instance_type = "local_gpu"
print("Instance type = " + instance_type)
%store -r train_dir
%store -r validation_dir
%store -r eval_dir
%store -r data_dir
###Output
_____no_output_____
###Markdown
2. 데이터 세트를 S3에 업로드
###Code
dataset_location = sagemaker_session.upload_data(path=data_dir, key_prefix='data/DEMO-cifar10')
display(dataset_location)
###Output
_____no_output_____
###Markdown
3. 모델 훈련 준비
###Code
import os
import subprocess
instance_type = "local_gpu"
# instance_type = "ml.p3.8xlarge"
job_name ='cifar10-horovod'
###Output
_____no_output_____
###Markdown
시스템의 이전 도커 컨테이너 삭제- 아래와 같은 명령어를 사용하여 저장 공간을 확보 합니다. 도커 컨테이너 모두 삭제
###Code
! df -h
# ! docker container prune -f
# ! rm -rf /tmp/tmp*
# ! df -h
###Output
_____no_output_____
###Markdown
도커 이미지 모두 삭제
###Code
# ! df -h
# ! docker image prune -f --all
# ! df -h
###Output
_____no_output_____
###Markdown
추가 용량 확보추가적인 용량 삭제가 필요하면 아래를 실행 하세요```rm -rf /tmp/tmp*``` 4. 로컬모드로 훈련- 현 실행 노트북 인스턴스에서 실행
###Code
def calculate_learning_rate(one_gpu_learning_rate, num_gpu, train_instance_count ):
total_gpu = num_gpu * train_instance_count
multi_gpu_learning_rate = one_gpu_learning_rate / total_gpu
print("multi_gpu_learning_rate: ", multi_gpu_learning_rate)
return multi_gpu_learning_rate
train_instance_type = 'ml.p3.16xlarge'
num_gpu = 8
train_instance_count = 1
one_gpu_learning_rate = 0.001
multi_gpu_learning_rate = calculate_learning_rate(one_gpu_learning_rate, num_gpu, train_instance_count )
hyperparameters = {
'epochs' : 10,
'learning-rate' : f"{multi_gpu_learning_rate}",
'print-interval' : 100,
'train-batch-size': 64,
'eval-batch-size': 512,
'validation-batch-size': 512,
}
from sagemaker.tensorflow import TensorFlow
job_name ='cifar10-sm-ddp'
estimator = TensorFlow(base_job_name= job_name,
entry_point='cifar10_tf2_sm_ddp.py',
source_dir='src',
role=role,
framework_version='2.4.1',
py_version='py37',
script_mode=True,
hyperparameters= hyperparameters,
train_instance_count=1, # 변경
train_instance_type='local_gpu',
distribution={"smdistributed": {"dataparallel": {"enabled": True}}},
debugger_hook_config=False,
)
estimator.fit({'train':'{}/train'.format(dataset_location),
'validation':'{}/validation'.format(dataset_location),
'eval':'{}/eval'.format(dataset_location)})
###Output
train_instance_type has been renamed in sagemaker>=2.
See: https://sagemaker.readthedocs.io/en/stable/v2.html for details.
train_instance_count has been renamed in sagemaker>=2.
See: https://sagemaker.readthedocs.io/en/stable/v2.html for details.
train_instance_type has been renamed in sagemaker>=2.
See: https://sagemaker.readthedocs.io/en/stable/v2.html for details.
###Markdown
로컬모드에서 도커 이미지 다운로드 된 것을 확인
###Code
! docker image ls
###Output
REPOSITORY TAG IMAGE ID CREATED SIZE
763104351884.dkr.ecr.us-east-1.amazonaws.com/tensorflow-training 2.4.1-gpu-py37 8467bc1c5070 5 months ago 8.91GB
###Markdown
5. 호스트 모드로 훈련 multi_gpu_learning_rate- GPU 의 개수, Batch Size, Epoch 당 배치 수 에 따라 튜닝이 필요한 수치 입니다. 여기서는 예시로 사용한 것이기에, 실제 사용시에 적절하게 튜닝을 해주시기 바랍니다.
###Code
train_instance_type = 'ml.p3.16xlarge'
num_gpu = 8
train_instance_count = 2
one_gpu_learning_rate = 0.001
multi_gpu_learning_rate = calculate_learning_rate(one_gpu_learning_rate, num_gpu, train_instance_count )
from sagemaker.tensorflow import TensorFlow
hyperparameters = {
'epochs' : 50,
'learning-rate' : f"{multi_gpu_learning_rate}",
'print-interval' : 100,
'train-batch-size': 64,
'eval-batch-size': 512,
'validation-batch-size': 512,
}
job_name ='cifar10-sm-ddp'
ddp_estimator = TensorFlow(base_job_name= job_name,
entry_point='cifar10_tf2_sm_ddp.py',
source_dir='src',
role=role,
framework_version='2.4.1',
py_version='py37',
script_mode=True,
hyperparameters= hyperparameters,
train_instance_count=train_instance_count, # 변경
train_instance_type=train_instance_type,
distribution={"smdistributed": {"dataparallel": {"enabled": True}}},
debugger_hook_config=False,
)
ddp_estimator.fit({'train':'{}/train'.format(dataset_location),
'validation':'{}/validation'.format(dataset_location),
'eval':'{}/eval'.format(dataset_location)}, wait=False)
ddp_estimator.logs()
###Output
2021-10-10 05:27:45 Starting - Starting the training job...
2021-10-10 05:27:48 Starting - Launching requested ML instancesProfilerReport-1633843665: InProgress
.........
2021-10-10 05:29:38 Starting - Preparing the instances for training.........
2021-10-10 05:31:06 Downloading - Downloading input data...
2021-10-10 05:31:39 Training - Downloading the training image..............[34m2021-10-10 05:33:57.516542: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m2021-10-10 05:33:57.523293: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:105] SageMaker Profiler is not enabled. The timeline writer thread will not be started, future recorded events will be dropped.[0m
[34m2021-10-10 05:33:57.641516: I tensorflow/stream_executor/platform/default/dso_loader.cc:49] Successfully opened dynamic library libcudart.so.11.0[0m
[34m2021-10-10 05:33:57.760163: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m2021-10-10 05:34:01,991 sagemaker-training-toolkit INFO Imported framework sagemaker_tensorflow_container.training[0m
2021-10-10 05:34:20 Training - Training image download completed. Training in progress.[34m2021-10-10 05:34:07.161161: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m2021-10-10 05:34:07.165720: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:105] SageMaker Profiler is not enabled. The timeline writer thread will not be started, future recorded events will be dropped.[0m
[34m2021-10-10 05:34:07.253622: I tensorflow/stream_executor/platform/default/dso_loader.cc:49] Successfully opened dynamic library libcudart.so.11.0[0m
[34m2021-10-10 05:34:07.350145: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[35m2021-10-10 05:34:09,030 sagemaker-training-toolkit INFO Starting MPI run as worker node.[0m
[35m2021-10-10 05:34:09,030 sagemaker-training-toolkit INFO Waiting for MPI Master to create SSH daemon.[0m
[35m2021-10-10 05:34:09,032 sagemaker-training-toolkit INFO Cannot connect to host algo-1[0m
[35m2021-10-10 05:34:09,032 sagemaker-training-toolkit INFO Connection failed with exception:
[Errno None] Unable to connect to port 22 on 10.0.242.34[0m
[35m2021-10-10 05:34:10,034 sagemaker-training-toolkit INFO Cannot connect to host algo-1[0m
[35m2021-10-10 05:34:10,034 sagemaker-training-toolkit INFO Connection failed with exception:
[Errno None] Unable to connect to port 22 on 10.0.242.34[0m
[35m2021-10-10 05:34:11,036 sagemaker-training-toolkit INFO Cannot connect to host algo-1[0m
[35m2021-10-10 05:34:11,036 sagemaker-training-toolkit INFO Connection failed with exception:
[Errno None] Unable to connect to port 22 on 10.0.242.34[0m
[34m2021-10-10 05:34:10,985 sagemaker-training-toolkit INFO Imported framework sagemaker_tensorflow_container.training[0m
[34m2021-10-10 05:34:11,666 sagemaker-training-toolkit INFO Starting MPI run as worker node.[0m
[34m2021-10-10 05:34:11,666 sagemaker-training-toolkit INFO Creating SSH daemon.[0m
[34m2021-10-10 05:34:11,675 sagemaker-training-toolkit INFO Waiting for MPI workers to establish their SSH connections[0m
[34m2021-10-10 05:34:11,677 sagemaker-training-toolkit INFO Cannot connect to host algo-2 at port 22. Retrying...[0m
[34m2021-10-10 05:34:11,678 sagemaker-training-toolkit INFO Connection closed[0m
[35m2021-10-10 05:34:12,045 paramiko.transport INFO Connected (version 2.0, client OpenSSH_7.6p1)[0m
[35m2021-10-10 05:34:12,122 paramiko.transport INFO Authentication (publickey) successful![0m
[35m2021-10-10 05:34:12,122 sagemaker-training-toolkit INFO Can connect to host algo-1[0m
[35m2021-10-10 05:34:12,123 sagemaker-training-toolkit INFO MPI Master online, creating SSH daemon.[0m
[35m2021-10-10 05:34:12,123 sagemaker-training-toolkit INFO Writing environment variables to /etc/environment for the MPI process.[0m
[35m2021-10-10 05:34:12,133 sagemaker-training-toolkit INFO Waiting for MPI process to finish.[0m
[34m2021-10-10 05:34:12,687 paramiko.transport INFO Connected (version 2.0, client OpenSSH_7.6p1)[0m
[34m2021-10-10 05:34:12,760 paramiko.transport INFO Authentication (publickey) successful![0m
[34m2021-10-10 05:34:12,761 sagemaker-training-toolkit INFO Can connect to host algo-2 at port 22[0m
[34m2021-10-10 05:34:12,761 sagemaker-training-toolkit INFO Connection closed[0m
[34m2021-10-10 05:34:12,761 sagemaker-training-toolkit INFO Worker algo-2 available for communication[0m
[34m2021-10-10 05:34:12,761 sagemaker-training-toolkit INFO Network interface name: eth0[0m
[34m2021-10-10 05:34:12,761 sagemaker-training-toolkit INFO Host: ['algo-1', 'algo-2'][0m
[34m2021-10-10 05:34:12,762 sagemaker-training-toolkit INFO instance type: ml.p3.16xlarge[0m
[34m2021-10-10 05:34:12,852 sagemaker-training-toolkit INFO Invoking user script
[0m
[34mTraining Env:
[0m
[34m{
"additional_framework_parameters": {
"sagemaker_distributed_dataparallel_enabled": true,
"sagemaker_instance_type": "ml.p3.16xlarge",
"sagemaker_distributed_dataparallel_custom_mpi_options": ""
},
"channel_input_dirs": {
"eval": "/opt/ml/input/data/eval",
"validation": "/opt/ml/input/data/validation",
"train": "/opt/ml/input/data/train"
},
"current_host": "algo-1",
"framework_module": "sagemaker_tensorflow_container.training:main",
"hosts": [
"algo-1",
"algo-2"
],
"hyperparameters": {
"validation-batch-size": 512,
"learning-rate": "6.25e-05",
"print-interval": 100,
"model_dir": "s3://sagemaker-us-east-1-057716757052/cifar10-sm-ddp-2021-10-10-05-27-44-881/model",
"train-batch-size": 64,
"epochs": 50,
"eval-batch-size": 512
},
"input_config_dir": "/opt/ml/input/config",
"input_data_config": {
"eval": {
"TrainingInputMode": "File",
"S3DistributionType": "FullyReplicated",
"RecordWrapperType": "None"
},
"validation": {
"TrainingInputMode": "File",
"S3DistributionType": "FullyReplicated",
"RecordWrapperType": "None"
},
"train": {
"TrainingInputMode": "File",
"S3DistributionType": "FullyReplicated",
"RecordWrapperType": "None"
}
},
"input_dir": "/opt/ml/input",
"is_master": true,
"job_name": "cifar10-sm-ddp-2021-10-10-05-27-44-881",
"log_level": 20,
"master_hostname": "algo-1",
"model_dir": "/opt/ml/model",
"module_dir": "s3://sagemaker-us-east-1-057716757052/cifar10-sm-ddp-2021-10-10-05-27-44-881/source/sourcedir.tar.gz",
"module_name": "cifar10_tf2_sm_ddp",
"network_interface_name": "eth0",
"num_cpus": 64,
"num_gpus": 8,
"output_data_dir": "/opt/ml/output/data",
"output_dir": "/opt/ml/output",
"output_intermediate_dir": "/opt/ml/output/intermediate",
"resource_config": {
"current_host": "algo-1",
"hosts": [
"algo-1",
"algo-2"
],
"network_interface_name": "eth0"
},
"user_entry_point": "cifar10_tf2_sm_ddp.py"[0m
[34m}
[0m
[34mEnvironment variables:
[0m
[34mSM_HOSTS=["algo-1","algo-2"][0m
[34mSM_NETWORK_INTERFACE_NAME=eth0[0m
[34mSM_HPS={"epochs":50,"eval-batch-size":512,"learning-rate":"6.25e-05","model_dir":"s3://sagemaker-us-east-1-057716757052/cifar10-sm-ddp-2021-10-10-05-27-44-881/model","print-interval":100,"train-batch-size":64,"validation-batch-size":512}[0m
[34mSM_USER_ENTRY_POINT=cifar10_tf2_sm_ddp.py[0m
[34mSM_FRAMEWORK_PARAMS={"sagemaker_distributed_dataparallel_custom_mpi_options":"","sagemaker_distributed_dataparallel_enabled":true,"sagemaker_instance_type":"ml.p3.16xlarge"}[0m
[34mSM_RESOURCE_CONFIG={"current_host":"algo-1","hosts":["algo-1","algo-2"],"network_interface_name":"eth0"}[0m
[34mSM_INPUT_DATA_CONFIG={"eval":{"RecordWrapperType":"None","S3DistributionType":"FullyReplicated","TrainingInputMode":"File"},"train":{"RecordWrapperType":"None","S3DistributionType":"FullyReplicated","TrainingInputMode":"File"},"validation":{"RecordWrapperType":"None","S3DistributionType":"FullyReplicated","TrainingInputMode":"File"}}[0m
[34mSM_OUTPUT_DATA_DIR=/opt/ml/output/data[0m
[34mSM_CHANNELS=["eval","train","validation"][0m
[34mSM_CURRENT_HOST=algo-1[0m
[34mSM_MODULE_NAME=cifar10_tf2_sm_ddp[0m
[34mSM_LOG_LEVEL=20[0m
[34mSM_FRAMEWORK_MODULE=sagemaker_tensorflow_container.training:main[0m
[34mSM_INPUT_DIR=/opt/ml/input[0m
[34mSM_INPUT_CONFIG_DIR=/opt/ml/input/config[0m
[34mSM_OUTPUT_DIR=/opt/ml/output[0m
[34mSM_NUM_CPUS=64[0m
[34mSM_NUM_GPUS=8[0m
[34mSM_MODEL_DIR=/opt/ml/model[0m
[34mSM_MODULE_DIR=s3://sagemaker-us-east-1-057716757052/cifar10-sm-ddp-2021-10-10-05-27-44-881/source/sourcedir.tar.gz[0m
[34mSM_TRAINING_ENV={"additional_framework_parameters":{"sagemaker_distributed_dataparallel_custom_mpi_options":"","sagemaker_distributed_dataparallel_enabled":true,"sagemaker_instance_type":"ml.p3.16xlarge"},"channel_input_dirs":{"eval":"/opt/ml/input/data/eval","train":"/opt/ml/input/data/train","validation":"/opt/ml/input/data/validation"},"current_host":"algo-1","framework_module":"sagemaker_tensorflow_container.training:main","hosts":["algo-1","algo-2"],"hyperparameters":{"epochs":50,"eval-batch-size":512,"learning-rate":"6.25e-05","model_dir":"s3://sagemaker-us-east-1-057716757052/cifar10-sm-ddp-2021-10-10-05-27-44-881/model","print-interval":100,"train-batch-size":64,"validation-batch-size":512},"input_config_dir":"/opt/ml/input/config","input_data_config":{"eval":{"RecordWrapperType":"None","S3DistributionType":"FullyReplicated","TrainingInputMode":"File"},"train":{"RecordWrapperType":"None","S3DistributionType":"FullyReplicated","TrainingInputMode":"File"},"validation":{"RecordWrapperType":"None","S3DistributionType":"FullyReplicated","TrainingInputMode":"File"}},"input_dir":"/opt/ml/input","is_master":true,"job_name":"cifar10-sm-ddp-2021-10-10-05-27-44-881","log_level":20,"master_hostname":"algo-1","model_dir":"/opt/ml/model","module_dir":"s3://sagemaker-us-east-1-057716757052/cifar10-sm-ddp-2021-10-10-05-27-44-881/source/sourcedir.tar.gz","module_name":"cifar10_tf2_sm_ddp","network_interface_name":"eth0","num_cpus":64,"num_gpus":8,"output_data_dir":"/opt/ml/output/data","output_dir":"/opt/ml/output","output_intermediate_dir":"/opt/ml/output/intermediate","resource_config":{"current_host":"algo-1","hosts":["algo-1","algo-2"],"network_interface_name":"eth0"},"user_entry_point":"cifar10_tf2_sm_ddp.py"}[0m
[34mSM_USER_ARGS=["--epochs","50","--eval-batch-size","512","--learning-rate","6.25e-05","--model_dir","s3://sagemaker-us-east-1-057716757052/cifar10-sm-ddp-2021-10-10-05-27-44-881/model","--print-interval","100","--train-batch-size","64","--validation-batch-size","512"][0m
[34mSM_OUTPUT_INTERMEDIATE_DIR=/opt/ml/output/intermediate[0m
[34mSM_CHANNEL_EVAL=/opt/ml/input/data/eval[0m
[34mSM_CHANNEL_VALIDATION=/opt/ml/input/data/validation[0m
[34mSM_CHANNEL_TRAIN=/opt/ml/input/data/train[0m
[34mSM_HP_VALIDATION-BATCH-SIZE=512[0m
[34mSM_HP_LEARNING-RATE=6.25e-05[0m
[34mSM_HP_PRINT-INTERVAL=100[0m
[34mSM_HP_MODEL_DIR=s3://sagemaker-us-east-1-057716757052/cifar10-sm-ddp-2021-10-10-05-27-44-881/model[0m
[34mSM_HP_TRAIN-BATCH-SIZE=64[0m
[34mSM_HP_EPOCHS=50[0m
[34mSM_HP_EVAL-BATCH-SIZE=512[0m
[34mPYTHONPATH=/opt/ml/code:/usr/local/bin:/usr/local/lib/python37.zip:/usr/local/lib/python3.7:/usr/local/lib/python3.7/lib-dynload:/usr/local/lib/python3.7/site-packages
[0m
[34mInvoking script with the following command:
[0m
[34mmpirun --host algo-1:8,algo-2:8 -np 16 --allow-run-as-root --tag-output --oversubscribe -mca btl_tcp_if_include eth0 -mca oob_tcp_if_include eth0 -mca plm_rsh_no_tree_spawn 1 -mca pml ob1 -mca btl ^openib -mca orte_abort_on_non_zero_status 1 -mca btl_vader_single_copy_mechanism none -mca plm_rsh_num_concurrent 2 -x NCCL_SOCKET_IFNAME=eth0 -x NCCL_DEBUG=INFO -x LD_LIBRARY_PATH -x PATH -x SMDATAPARALLEL_USE_HOMOGENEOUS=1 -x FI_PROVIDER=efa -x RDMAV_FORK_SAFE=1 -x LD_PRELOAD=/usr/local/lib/python3.7/site-packages/gethostname.cpython-37m-x86_64-linux-gnu.so -x SMDATAPARALLEL_SERVER_ADDR=algo-1 -x SMDATAPARALLEL_SERVER_PORT=7592 -x SAGEMAKER_INSTANCE_TYPE=ml.p3.16xlarge smddprun /usr/local/bin/python3.7 -m mpi4py cifar10_tf2_sm_ddp.py --epochs 50 --eval-batch-size 512 --learning-rate 6.25e-05 --model_dir s3://sagemaker-us-east-1-057716757052/cifar10-sm-ddp-2021-10-10-05-27-44-881/model --print-interval 100 --train-batch-size 64 --validation-batch-size 512
[0m
[35m2021-10-10 05:34:14,138 sagemaker-training-toolkit INFO Process[es]: [psutil.Process(pid=172, name='orted', status='disk-sleep', started='05:34:13')][0m
[35m2021-10-10 05:34:14,138 sagemaker-training-toolkit INFO Orted process found [psutil.Process(pid=172, name='orted', status='disk-sleep', started='05:34:13')][0m
[35m2021-10-10 05:34:14,139 sagemaker-training-toolkit INFO Waiting for orted process [psutil.Process(pid=172, name='orted', status='disk-sleep', started='05:34:13')][0m
[34m[1,3]<stdout>:tensorflow version: 2.4.1[0m
[34m[1,1]<stdout>:tensorflow version: 2.4.1[0m
[34m[1,10]<stdout>:tensorflow version: 2.4.1[0m
[34m[1,9]<stdout>:tensorflow version: 2.4.1[0m
[34m[1,7]<stdout>:tensorflow version: 2.4.1[0m
[34m[1,12]<stdout>:tensorflow version: 2.4.1[0m
[34m[1,6]<stdout>:tensorflow version: 2.4.1[0m
[34m[1,11]<stdout>:tensorflow version: 2.4.1[0m
[34m[1,13]<stdout>:tensorflow version: 2.4.1[0m
[34m[1,5]<stdout>:tensorflow version: 2.4.1[0m
[34m[1,4]<stdout>:tensorflow version: 2.4.1[0m
[34m[1,2]<stdout>:tensorflow version: 2.4.1[0m
[34m[1,15]<stdout>:tensorflow version: 2.4.1[0m
[34m[1,14]<stdout>:tensorflow version: 2.4.1[0m
[34m[1,8]<stdout>:tensorflow version: 2.4.1[0m
[34m[1,0]<stdout>:tensorflow version: 2.4.1[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO Bootstrap : Using [0]eth0:10.0.253.10<0>[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Bootstrap : Using [0]eth0:10.0.242.34<0>[0m
[34m[1,8]<stdout>:[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] find_ofi_provider:542 NCCL WARN NET/OFI Couldn't find any optimal provider[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO NET/IB : No device found.[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO NET/Socket : Using [0]eth0:10.0.253.10<0>[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO Using network Socket[0m
[34m[1,8]<stdout>:NCCL version 2.7.8+cuda11.0[0m
[34m[1,0]<stdout>:[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] find_ofi_provider:542 NCCL WARN NET/OFI Couldn't find any optimal provider[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO NET/IB : No device found.[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO NET/Socket : Using [0]eth0:10.0.242.34<0>[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Using network Socket[0m
[34m[1,0]<stdout>:NCCL version 2.7.8+cuda11.0[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO Bootstrap : Using [0]eth0:10.0.253.10<0>[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO Bootstrap : Using [0]eth0:10.0.253.10<0>[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO Bootstrap : Using [0]eth0:10.0.253.10<0>[0m
[34m[1,10]<stdout>:[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] find_ofi_provider:542 NCCL WARN NET/OFI Couldn't find any optimal provider[0m
[34m[1,11]<stdout>:[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] find_ofi_provider:542 NCCL WARN NET/OFI Couldn't find any optimal provider[0m
[34m[1,9]<stdout>:[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] find_ofi_provider:542 NCCL WARN NET/OFI Couldn't find any optimal provider[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO NET/IB : No device found.[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO NET/IB : No device found.[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO NET/IB : No device found.[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO NET/Socket : Using [0]eth0:10.0.253.10<0>[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO Using network Socket[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO NET/Socket : Using [0]eth0:10.0.253.10<0>[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO Using network Socket[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO NET/Socket : Using [0]eth0:10.0.253.10<0>[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO Using network Socket[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO Bootstrap : Using [0]eth0:10.0.242.34<0>[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO Bootstrap : Using [0]eth0:10.0.242.34<0>[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO Bootstrap : Using [0]eth0:10.0.242.34<0>[0m
[34m[1,2]<stdout>:[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] find_ofi_provider:542 NCCL WARN NET/OFI Couldn't find any optimal provider[0m
[34m[1,1]<stdout>:[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] find_ofi_provider:542 NCCL WARN NET/OFI Couldn't find any optimal provider[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO NET/IB : No device found.[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO NET/IB : No device found.[0m
[34m[1,3]<stdout>:[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] find_ofi_provider:542 NCCL WARN NET/OFI Couldn't find any optimal provider[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO NET/Socket : Using [0]eth0:10.0.242.34<0>[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO Using network Socket[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO NET/Socket : Using [0]eth0:10.0.242.34<0>[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO Using network Socket[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO NET/IB : No device found.[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO NET/Socket : Using [0]eth0:10.0.242.34<0>[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO Using network Socket[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO Bootstrap : Using [0]eth0:10.0.242.34<0>[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO Bootstrap : Using [0]eth0:10.0.242.34<0>[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO Bootstrap : Using [0]eth0:10.0.242.34<0>[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO Bootstrap : Using [0]eth0:10.0.242.34<0>[0m
[34m[1,6]<stdout>:[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] find_ofi_provider:542 NCCL WARN NET/OFI Couldn't find any optimal provider[0m
[34m[1,4]<stdout>:[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] find_ofi_provider:542 NCCL WARN NET/OFI Couldn't find any optimal provider[0m
[34m[1,5]<stdout>:[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] find_ofi_provider:542 NCCL WARN NET/OFI Couldn't find any optimal provider[0m
[34m[1,7]<stdout>:[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] find_ofi_provider:542 NCCL WARN NET/OFI Couldn't find any optimal provider[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO NET/IB : No device found.[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO NET/IB : No device found.[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO NET/IB : No device found.[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO NET/IB : No device found.[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO NET/Socket : Using [0]eth0:10.0.242.34<0>[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO Using network Socket[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO NET/Socket : Using [0]eth0:10.0.242.34<0>[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO Using network Socket[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO NET/Socket : Using [0]eth0:10.0.242.34<0>[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO Using network Socket[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO NET/Socket : Using [0]eth0:10.0.242.34<0>[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO Using network Socket[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO Bootstrap : Using [0]eth0:10.0.253.10<0>[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO Bootstrap : Using [0]eth0:10.0.253.10<0>[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO Bootstrap : Using [0]eth0:10.0.253.10<0>[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO Bootstrap : Using [0]eth0:10.0.253.10<0>[0m
[34m[1,14]<stdout>:[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] find_ofi_provider:542 NCCL WARN NET/OFI Couldn't find any optimal provider[0m
[34m[1,12]<stdout>:[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] find_ofi_provider:542 NCCL WARN NET/OFI Couldn't find any optimal provider[0m
[34m[1,13]<stdout>:[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] find_ofi_provider:542 NCCL WARN NET/OFI Couldn't find any optimal provider[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO NET/IB : No device found.[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO NET/IB : No device found.[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO NET/IB : No device found.[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO NET/Socket : Using [0]eth0:10.0.253.10<0>[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO Using network Socket[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO NET/Socket : Using [0]eth0:10.0.253.10<0>[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO Using network Socket[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO NET/Socket : Using [0]eth0:10.0.253.10<0>[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO Using network Socket[0m
[34m[1,15]<stdout>:[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] find_ofi_provider:542 NCCL WARN NET/OFI Couldn't find any optimal provider[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO NET/IB : No device found.[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO NET/Socket : Using [0]eth0:10.0.253.10<0>[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO Using network Socket[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO threadThresholds 8/8/64 | 64/8/64 | 8/8/64[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO Trees [0] 7/-1/-1->6->5|5->6->7/-1/-1 [1] 7/-1/-1->6->5|5->6->7/-1/-1 [2] 5/-1/-1->6->7|7->6->5/-1/-1 [3] 5/-1/-1->6->7|7->6->5/-1/-1 [4] 2/-1/-1->6->4|4->6->2/-1/-1 [5] 4/-1/-1->6->2|2->6->4/-1/-1 [6] 7/-1/-1->6->5|5->6->7/-1/-1 [7] 7/-1/-1->6->5|5->6->7/-1/-1 [8] 5/-1/-1->6->7|7->6->5/-1/-1 [9] 5/-1/-1->6->7|7->6->5/-1/-1 [10] 2/-1/-1->6->4|4->6->2/-1/-1 [11] 4/-1/-1->6->2|2->6->4/-1/-1[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO threadThresholds 8/8/64 | 64/8/64 | 8/8/64[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO Trees [0] -1/-1/-1->4->7|7->4->-1/-1/-1 [1] -1/-1/-1->4->7|7->4->-1/-1/-1 [2] 7/-1/-1->4->0|0->4->7/-1/-1 [3] 7/-1/-1->4->0|0->4->7/-1/-1 [4] 6/-1/-1->4->5|5->4->6/-1/-1 [5] 5/-1/-1->4->6|6->4->5/-1/-1 [6] -1/-1/-1->4->7|7->4->-1/-1/-1 [7] -1/-1/-1->4->7|7->4->-1/-1/-1 [8] 7/-1/-1->4->0|0->4->7/-1/-1 [9] 7/-1/-1->4->0|0->4->7/-1/-1 [10] 6/-1/-1->4->5|5->4->6/-1/-1 [11] 5/-1/-1->4->6|6->4->5/-1/-1[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO threadThresholds 8/8/64 | 64/8/64 | 8/8/64[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO Trees [0] 6/-1/-1->5->1|1->5->6/-1/-1 [1] 6/-1/-1->5->1|1->5->6/-1/-1 [2] 1/-1/-1->5->6|6->5->1/-1/-1 [3] 1/-1/-1->5->6|6->5->1/-1/-1 [4] 4/-1/-1->5->7|7->5->4/-1/-1 [5] 7/-1/-1->5->4|4->5->7/-1/-1 [6] 6/-1/-1->5->1|1->5->6/-1/-1 [7] 6/-1/-1->5->1|1->5->6/-1/-1 [8] 1/-1/-1->5->6|6->5->1/-1/-1 [9] 1/-1/-1->5->6|6->5->1/-1/-1 [10] 4/-1/-1->5->7|7->5->4/-1/-1 [11] 7/-1/-1->5->4|4->5->7/-1/-1[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Channel 00/12 : 0 3 2 1 5 6 7 4[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO threadThresholds 8/8/64 | 64/8/64 | 8/8/64[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO Trees [0] 4/-1/-1->7->6|6->7->4/-1/-1 [1] 4/-1/-1->7->6|6->7->4/-1/-1 [2] 6/-1/-1->7->4|4->7->6/-1/-1 [3] 6/-1/-1->7->4|4->7->6/-1/-1 [4] 5/-1/-1->7->3|3->7->5/-1/-1 [5] 3/-1/-1->7->5|5->7->3/-1/-1 [6] 4/-1/-1->7->6|6->7->4/-1/-1 [7] 4/-1/-1->7->6|6->7->4/-1/-1 [8] 6/-1/-1->7->4|4->7->6/-1/-1 [9] 6/-1/-1->7->4|4->7->6/-1/-1 [10] 5/-1/-1->7->3|3->7->5/-1/-1 [11] 3/-1/-1->7->5|5->7->3/-1/-1[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO threadThresholds 8/8/64 | 64/8/64 | 8/8/64[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Channel 01/12 : 0 3 2 1 5 6 7 4[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Channel 02/12 : 0 4 7 6 5 1 2 3[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Channel 03/12 : 0 4 7 6 5 1 2 3[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Channel 04/12 : 0 1 3 7 5 4 6 2[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO Trees [0] 5/-1/-1->1->2|2->1->5/-1/-1 [1] 5/-1/-1->1->2|2->1->5/-1/-1 [2] 2/-1/-1->1->5|5->1->2/-1/-1 [3] 2/-1/-1->1->5|5->1->2/-1/-1 [4] 3/-1/-1->1->0|0->1->3/-1/-1 [5] -1/-1/-1->1->3|3->1->-1/-1/-1 [6] 5/-1/-1->1->2|2->1->5/-1/-1 [7] 5/-1/-1->1->2|2->1->5/-1/-1 [8] 2/-1/-1->1->5|5->1->2/-1/-1 [9] 2/-1/-1->1->5|5->1->2/-1/-1 [10] 3/-1/-1->1->0|0->1->3/-1/-1 [11] -1/-1/-1->1->3|3->1->-1/-1/-1[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO threadThresholds 8/8/64 | 64/8/64 | 8/8/64[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Channel 05/12 : 0 2 6 4 5 7 3 1[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Channel 06/12 : 0 3 2 1 5 6 7 4[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Channel 07/12 : 0 3 2 1 5 6 7 4[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Channel 08/12 : 0 4 7 6 5 1 2 3[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO threadThresholds 8/8/64 | 64/8/64 | 8/8/64[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO Trees [0] 1/-1/-1->2->3|3->2->1/-1/-1 [1] 1/-1/-1->2->3|3->2->1/-1/-1 [2] 3/-1/-1->2->1|1->2->3/-1/-1 [3] 3/-1/-1->2->1|1->2->3/-1/-1 [4] -1/-1/-1->2->6|6->2->-1/-1/-1 [5] 6/-1/-1->2->0|0->2->6/-1/-1 [6] 1/-1/-1->2->3|3->2->1/-1/-1 [7] 1/-1/-1->2->3|3->2->1/-1/-1 [8] 3/-1/-1->2->1|1->2->3/-1/-1 [9] 3/-1/-1->2->1|1->2->3/-1/-1 [10] -1/-1/-1->2->6|6->2->-1/-1/-1 [11] 6/-1/-1->2->0|0->2->6/-1/-1[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Channel 09/12 : 0 4 7 6 5 1 2 3[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Channel 10/12 : 0 1 3 7 5 4 6 2[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Channel 11/12 : 0 2 6 4 5 7 3 1[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO Trees [0] 2/-1/-1->3->0|0->3->2/-1/-1 [1] 2/-1/-1->3->0|0->3->2/-1/-1 [2] -1/-1/-1->3->2|2->3->-1/-1/-1 [3] -1/-1/-1->3->2|2->3->-1/-1/-1 [4] 7/-1/-1->3->1|1->3->7/-1/-1 [5] 1/-1/-1->3->7|7->3->1/-1/-1 [6] 2/-1/-1->3->0|0->3->2/-1/-1 [7] 2/-1/-1->3->0|0->3->2/-1/-1 [8] -1/-1/-1->3->2|2->3->-1/-1/-1 [9] -1/-1/-1->3->2|2->3->-1/-1/-1 [10] 7/-1/-1->3->1|1->3->7/-1/-1 [11] 1/-1/-1->3->7|7->3->1/-1/-1[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO threadThresholds 8/8/64 | 64/8/64 | 8/8/64[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Trees [0] 3/-1/-1->0->-1|-1->0->3/-1/-1 [1] 3/-1/-1->0->-1|-1->0->3/-1/-1 [2] 4/-1/-1->0->-1|-1->0->4/-1/-1 [3] 4/-1/-1->0->-1|-1->0->4/-1/-1 [4] 1/-1/-1->0->-1|-1->0->1/-1/-1 [5] 2/-1/-1->0->-1|-1->0->2/-1/-1 [6] 3/-1/-1->0->-1|-1->0->3/-1/-1 [7] 3/-1/-1->0->-1|-1->0->3/-1/-1 [8] 4/-1/-1->0->-1|-1->0->4/-1/-1 [9] 4/-1/-1->0->-1|-1->0->4/-1/-1 [10] 1/-1/-1->0->-1|-1->0->1/-1/-1 [11] 2/-1/-1->0->-1|-1->0->2/-1/-1[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO Channel 00 : 5[1c0] -> 6[1d0] via P2P/IPC[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO Channel 00 : 4[1b0] -> 0[170] via P2P/IPC[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO Channel 00 : 6[1d0] -> 7[1e0] via P2P/IPC[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO Channel 00 : 7[1e0] -> 4[1b0] via P2P/IPC[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO Channel 00 : 1[180] -> 5[1c0] via P2P/IPC[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO Channel 00 : 2[190] -> 1[180] via P2P/IPC[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO Channel 00 : 3[1a0] -> 2[190] via P2P/IPC[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Channel 00 : 0[170] -> 3[1a0] via P2P/IPC[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO Channel 00 : 4[1b0] -> 7[1e0] via P2P/IPC[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO Channel 00 : 5[1c0] -> 1[180] via P2P/IPC[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO Channel 00 : 6[1d0] -> 5[1c0] via P2P/IPC[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO Channel 00 : 7[1e0] -> 6[1d0] via P2P/IPC[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO Channel 00 : 3[1a0] -> 0[170] via P2P/IPC[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO Channel 00 : 1[180] -> 2[190] via P2P/IPC[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO Channel 00 : 2[190] -> 3[1a0] via P2P/IPC[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO threadThresholds 8/8/64 | 64/8/64 | 8/8/64[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO Trees [0] 6/-1/-1->5->1|1->5->6/-1/-1 [1] 6/-1/-1->5->1|1->5->6/-1/-1 [2] 1/-1/-1->5->6|6->5->1/-1/-1 [3] 1/-1/-1->5->6|6->5->1/-1/-1 [4] 4/-1/-1->5->7|7->5->4/-1/-1 [5] 7/-1/-1->5->4|4->5->7/-1/-1 [6] 6/-1/-1->5->1|1->5->6/-1/-1 [7] 6/-1/-1->5->1|1->5->6/-1/-1 [8] 1/-1/-1->5->6|6->5->1/-1/-1 [9] 1/-1/-1->5->6|6->5->1/-1/-1 [10] 4/-1/-1->5->7|7->5->4/-1/-1 [11] 7/-1/-1->5->4|4->5->7/-1/-1[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO threadThresholds 8/8/64 | 64/8/64 | 8/8/64[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO Trees [0] -1/-1/-1->4->7|7->4->-1/-1/-1 [1] -1/-1/-1->4->7|7->4->-1/-1/-1 [2] 7/-1/-1->4->0|0->4->7/-1/-1 [3] 7/-1/-1->4->0|0->4->7/-1/-1 [4] 6/-1/-1->4->5|5->4->6/-1/-1 [5] 5/-1/-1->4->6|6->4->5/-1/-1 [6] -1/-1/-1->4->7|7->4->-1/-1/-1 [7] -1/-1/-1->4->7|7->4->-1/-1/-1 [8] 7/-1/-1->4->0|0->4->7/-1/-1 [9] 7/-1/-1->4->0|0->4->7/-1/-1 [10] 6/-1/-1->4->5|5->4->6/-1/-1 [11] 5/-1/-1->4->6|6->4->5/-1/-1[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO threadThresholds 8/8/64 | 64/8/64 | 8/8/64[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO Trees [0] 4/-1/-1->7->6|6->7->4/-1/-1 [1] 4/-1/-1->7->6|6->7->4/-1/-1 [2] 6/-1/-1->7->4|4->7->6/-1/-1 [3] 6/-1/-1->7->4|4->7->6/-1/-1 [4] 5/-1/-1->7->3|3->7->5/-1/-1 [5] 3/-1/-1->7->5|5->7->3/-1/-1 [6] 4/-1/-1->7->6|6->7->4/-1/-1 [7] 4/-1/-1->7->6|6->7->4/-1/-1 [8] 6/-1/-1->7->4|4->7->6/-1/-1 [9] 6/-1/-1->7->4|4->7->6/-1/-1 [10] 5/-1/-1->7->3|3->7->5/-1/-1 [11] 3/-1/-1->7->5|5->7->3/-1/-1[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO threadThresholds 8/8/64 | 64/8/64 | 8/8/64[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO Trees [0] 7/-1/-1->6->5|5->6->7/-1/-1 [1] 7/-1/-1->6->5|5->6->7/-1/-1 [2] 5/-1/-1->6->7|7->6->5/-1/-1 [3] 5/-1/-1->6->7|7->6->5/-1/-1 [4] 2/-1/-1->6->4|4->6->2/-1/-1 [5] 4/-1/-1->6->2|2->6->4/-1/-1 [6] 7/-1/-1->6->5|5->6->7/-1/-1 [7] 7/-1/-1->6->5|5->6->7/-1/-1 [8] 5/-1/-1->6->7|7->6->5/-1/-1 [9] 5/-1/-1->6->7|7->6->5/-1/-1 [10] 2/-1/-1->6->4|4->6->2/-1/-1 [11] 4/-1/-1->6->2|2->6->4/-1/-1[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO Channel 00/12 : 0 3 2 1 5 6 7 4[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO Channel 01/12 : 0 3 2 1 5 6 7 4[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO Channel 02/12 : 0 4 7 6 5 1 2 3[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO Channel 03/12 : 0 4 7 6 5 1 2 3[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO threadThresholds 8/8/64 | 64/8/64 | 8/8/64[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO Channel 04/12 : 0 1 3 7 5 4 6 2[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO Channel 05/12 : 0 2 6 4 5 7 3 1[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO Trees [0] 5/-1/-1->1->2|2->1->5/-1/-1 [1] 5/-1/-1->1->2|2->1->5/-1/-1 [2] 2/-1/-1->1->5|5->1->2/-1/-1 [3] 2/-1/-1->1->5|5->1->2/-1/-1 [4] 3/-1/-1->1->0|0->1->3/-1/-1 [5] -1/-1/-1->1->3|3->1->-1/-1/-1 [6] 5/-1/-1->1->2|2->1->5/-1/-1 [7] 5/-1/-1->1->2|2->1->5/-1/-1 [8] 2/-1/-1->1->5|5->1->2/-1/-1 [9] 2/-1/-1->1->5|5->1->2/-1/-1 [10] 3/-1/-1->1->0|0->1->3/-1/-1 [11] -1/-1/-1->1->3|3->1->-1/-1/-1[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO threadThresholds 8/8/64 | 64/8/64 | 8/8/64[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO threadThresholds 8/8/64 | 64/8/64 | 8/8/64[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO Channel 06/12 : 0 3 2 1 5 6 7 4[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO Channel 07/12 : 0 3 2 1 5 6 7 4[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO Channel 08/12 : 0 4 7 6 5 1 2 3[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO Trees [0] 2/-1/-1->3->0|0->3->2/-1/-1 [1] 2/-1/-1->3->0|0->3->2/-1/-1 [2] -1/-1/-1->3->2|2->3->-1/-1/-1 [3] -1/-1/-1->3->2|2->3->-1/-1/-1 [4] 7/-1/-1->3->1|1->3->7/-1/-1 [5] 1/-1/-1->3->7|7->3->1/-1/-1 [6] 2/-1/-1->3->0|0->3->2/-1/-1 [7] 2/-1/-1->3->0|0->3->2/-1/-1 [8] -1/-1/-1->3->2|2->3->-1/-1/-1 [9] -1/-1/-1->3->2|2->3->-1/-1/-1 [10] 7/-1/-1->3->1|1->3->7/-1/-1 [11] 1/-1/-1->3->7|7->3->1/-1/-1[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO Trees [0] 1/-1/-1->2->3|3->2->1/-1/-1 [1] 1/-1/-1->2->3|3->2->1/-1/-1 [2] 3/-1/-1->2->1|1->2->3/-1/-1 [3] 3/-1/-1->2->1|1->2->3/-1/-1 [4] -1/-1/-1->2->6|6->2->-1/-1/-1 [5] 6/-1/-1->2->0|0->2->6/-1/-1 [6] 1/-1/-1->2->3|3->2->1/-1/-1 [7] 1/-1/-1->2->3|3->2->1/-1/-1 [8] 3/-1/-1->2->1|1->2->3/-1/-1 [9] 3/-1/-1->2->1|1->2->3/-1/-1 [10] -1/-1/-1->2->6|6->2->-1/-1/-1 [11] 6/-1/-1->2->0|0->2->6/-1/-1[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO Channel 09/12 : 0 4 7 6 5 1 2 3[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO Channel 10/12 : 0 1 3 7 5 4 6 2[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO Channel 11/12 : 0 2 6 4 5 7 3 1[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO threadThresholds 8/8/64 | 64/8/64 | 8/8/64[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO Trees [0] 3/-1/-1->0->-1|-1->0->3/-1/-1 [1] 3/-1/-1->0->-1|-1->0->3/-1/-1 [2] 4/-1/-1->0->-1|-1->0->4/-1/-1 [3] 4/-1/-1->0->-1|-1->0->4/-1/-1 [4] 1/-1/-1->0->-1|-1->0->1/-1/-1 [5] 2/-1/-1->0->-1|-1->0->2/-1/-1 [6] 3/-1/-1->0->-1|-1->0->3/-1/-1 [7] 3/-1/-1->0->-1|-1->0->3/-1/-1 [8] 4/-1/-1->0->-1|-1->0->4/-1/-1 [9] 4/-1/-1->0->-1|-1->0->4/-1/-1 [10] 1/-1/-1->0->-1|-1->0->1/-1/-1 [11] 2/-1/-1->0->-1|-1->0->2/-1/-1[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO Channel 01 : 4[1b0] -> 0[170] via P2P/IPC[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Channel 01 : 0[170] -> 3[1a0] via P2P/IPC[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO Channel 01 : 5[1c0] -> 6[1d0] via P2P/IPC[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO Channel 01 : 6[1d0] -> 7[1e0] via P2P/IPC[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO Channel 01 : 7[1e0] -> 4[1b0] via P2P/IPC[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO Channel 01 : 2[190] -> 1[180] via P2P/IPC[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO Channel 01 : 1[180] -> 5[1c0] via P2P/IPC[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO Channel 01 : 3[1a0] -> 2[190] via P2P/IPC[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO Channel 00 : 4[1b0] -> 0[170] via P2P/IPC[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO Channel 00 : 5[1c0] -> 6[1d0] via P2P/IPC[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO Channel 00 : 6[1d0] -> 7[1e0] via P2P/IPC[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO Channel 00 : 1[180] -> 5[1c0] via P2P/IPC[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO Channel 00 : 7[1e0] -> 4[1b0] via P2P/IPC[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO Channel 00 : 2[190] -> 1[180] via P2P/IPC[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO Channel 00 : 3[1a0] -> 2[190] via P2P/IPC[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO Channel 00 : 0[170] -> 3[1a0] via P2P/IPC[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO Channel 01 : 4[1b0] -> 7[1e0] via P2P/IPC[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO Channel 01 : 5[1c0] -> 1[180] via P2P/IPC[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO Channel 01 : 6[1d0] -> 5[1c0] via P2P/IPC[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO Channel 01 : 7[1e0] -> 6[1d0] via P2P/IPC[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO Channel 01 : 2[190] -> 3[1a0] via P2P/IPC[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO Channel 01 : 1[180] -> 2[190] via P2P/IPC[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO Channel 01 : 3[1a0] -> 0[170] via P2P/IPC[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO Channel 00 : 4[1b0] -> 7[1e0] via P2P/IPC[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO Channel 00 : 5[1c0] -> 1[180] via P2P/IPC[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO Channel 00 : 6[1d0] -> 5[1c0] via P2P/IPC[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO Channel 00 : 7[1e0] -> 6[1d0] via P2P/IPC[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO Channel 00 : 1[180] -> 2[190] via P2P/IPC[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO Channel 00 : 2[190] -> 3[1a0] via P2P/IPC[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO Channel 00 : 3[1a0] -> 0[170] via P2P/IPC[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO Channel 02 : 4[1b0] -> 7[1e0] via P2P/IPC[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO Channel 02 : 5[1c0] -> 1[180] via P2P/IPC[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Channel 02 : 0[170] -> 4[1b0] via P2P/IPC[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO Channel 02 : 6[1d0] -> 5[1c0] via P2P/IPC[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO Channel 01 : 4[1b0] -> 0[170] via P2P/IPC[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO Channel 02 : 2[190] -> 3[1a0] via P2P/IPC[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO Channel 02 : 7[1e0] -> 6[1d0] via P2P/IPC[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO Channel 02 : 1[180] -> 2[190] via P2P/IPC[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO Channel 02 : 3[1a0] -> 0[170] via P2P/IPC[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO Channel 01 : 0[170] -> 3[1a0] via P2P/IPC[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO Channel 01 : 5[1c0] -> 6[1d0] via P2P/IPC[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO Channel 01 : 6[1d0] -> 7[1e0] via P2P/IPC[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO Channel 01 : 7[1e0] -> 4[1b0] via P2P/IPC[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO Channel 01 : 1[180] -> 5[1c0] via P2P/IPC[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO Channel 01 : 2[190] -> 1[180] via P2P/IPC[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO Channel 01 : 3[1a0] -> 2[190] via P2P/IPC[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO Channel 02 : 4[1b0] -> 0[170] via P2P/IPC[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO Channel 02 : 3[1a0] -> 2[190] via P2P/IPC[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO Channel 02 : 5[1c0] -> 6[1d0] via P2P/IPC[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO Channel 02 : 6[1d0] -> 7[1e0] via P2P/IPC[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO Channel 01 : 4[1b0] -> 7[1e0] via P2P/IPC[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO Channel 02 : 2[190] -> 1[180] via P2P/IPC[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO Channel 02 : 7[1e0] -> 4[1b0] via P2P/IPC[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO Channel 02 : 1[180] -> 5[1c0] via P2P/IPC[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO Channel 01 : 5[1c0] -> 1[180] via P2P/IPC[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO Channel 01 : 6[1d0] -> 5[1c0] via P2P/IPC[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO Channel 01 : 1[180] -> 2[190] via P2P/IPC[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO Channel 01 : 7[1e0] -> 6[1d0] via P2P/IPC[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO Channel 01 : 2[190] -> 3[1a0] via P2P/IPC[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO Channel 01 : 3[1a0] -> 0[170] via P2P/IPC[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Channel 03 : 0[170] -> 4[1b0] via P2P/IPC[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO Channel 03 : 3[1a0] -> 0[170] via P2P/IPC[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO Channel 03 : 4[1b0] -> 7[1e0] via P2P/IPC[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO Channel 02 : 4[1b0] -> 7[1e0] via P2P/IPC[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO Channel 03 : 5[1c0] -> 1[180] via P2P/IPC[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO Channel 03 : 6[1d0] -> 5[1c0] via P2P/IPC[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO Channel 03 : 7[1e0] -> 6[1d0] via P2P/IPC[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO Channel 03 : 2[190] -> 3[1a0] via P2P/IPC[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO Channel 03 : 1[180] -> 2[190] via P2P/IPC[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO Channel 02 : 0[170] -> 4[1b0] via P2P/IPC[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO Channel 02 : 5[1c0] -> 1[180] via P2P/IPC[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO Channel 02 : 6[1d0] -> 5[1c0] via P2P/IPC[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO Channel 02 : 7[1e0] -> 6[1d0] via P2P/IPC[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO Channel 02 : 1[180] -> 2[190] via P2P/IPC[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO Channel 02 : 2[190] -> 3[1a0] via P2P/IPC[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO Channel 02 : 3[1a0] -> 0[170] via P2P/IPC[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO Channel 03 : 3[1a0] -> 2[190] via P2P/IPC[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO Channel 03 : 4[1b0] -> 0[170] via P2P/IPC[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO Channel 03 : 6[1d0] -> 7[1e0] via P2P/IPC[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO Channel 03 : 5[1c0] -> 6[1d0] via P2P/IPC[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO Channel 03 : 7[1e0] -> 4[1b0] via P2P/IPC[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO Channel 03 : 2[190] -> 1[180] via P2P/IPC[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO Channel 03 : 1[180] -> 5[1c0] via P2P/IPC[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO Channel 02 : 4[1b0] -> 0[170] via P2P/IPC[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO Channel 02 : 3[1a0] -> 2[190] via P2P/IPC[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO Channel 02 : 5[1c0] -> 6[1d0] via P2P/IPC[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO Channel 02 : 6[1d0] -> 7[1e0] via P2P/IPC[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO Channel 02 : 7[1e0] -> 4[1b0] via P2P/IPC[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO Channel 02 : 1[180] -> 5[1c0] via P2P/IPC[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO Channel 02 : 2[190] -> 1[180] via P2P/IPC[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO Channel 04 : 3[1a0] -> 7[1e0] via P2P/IPC[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Channel 04 : 0[170] -> 1[180] via P2P/IPC[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO Channel 04 : 4[1b0] -> 6[1d0] via P2P/IPC[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO Channel 04 : 5[1c0] -> 4[1b0] via P2P/IPC[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO Channel 04 : 6[1d0] -> 2[190] via P2P/IPC[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO Channel 03 : 0[170] -> 4[1b0] via P2P/IPC[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO Channel 04 : 7[1e0] -> 5[1c0] via P2P/IPC[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO Channel 03 : 3[1a0] -> 0[170] via P2P/IPC[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO Channel 04 : 2[190] -> 0[170] via P2P/IPC[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO Channel 04 : 1[180] -> 3[1a0] via P2P/IPC[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO Channel 03 : 4[1b0] -> 7[1e0] via P2P/IPC[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO Channel 03 : 5[1c0] -> 1[180] via P2P/IPC[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO Channel 03 : 6[1d0] -> 5[1c0] via P2P/IPC[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO Channel 03 : 7[1e0] -> 6[1d0] via P2P/IPC[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO Channel 03 : 1[180] -> 2[190] via P2P/IPC[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO Channel 03 : 2[190] -> 3[1a0] via P2P/IPC[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO Channel 04 : 2[190] -> 6[1d0] via P2P/IPC[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO Channel 04 : 4[1b0] -> 5[1c0] via P2P/IPC[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO Channel 04 : 3[1a0] -> 1[180] via P2P/IPC[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO Channel 03 : 3[1a0] -> 2[190] via P2P/IPC[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO Channel 04 : 5[1c0] -> 7[1e0] via P2P/IPC[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO Channel 04 : 6[1d0] -> 4[1b0] via P2P/IPC[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO Channel 04 : 7[1e0] -> 3[1a0] via P2P/IPC[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO Channel 03 : 4[1b0] -> 0[170] via P2P/IPC[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO Channel 04 : 1[180] -> 0[170] via P2P/IPC[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO Channel 03 : 5[1c0] -> 6[1d0] via P2P/IPC[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO Channel 03 : 6[1d0] -> 7[1e0] via P2P/IPC[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO Channel 03 : 7[1e0] -> 4[1b0] via P2P/IPC[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO Channel 03 : 1[180] -> 5[1c0] via P2P/IPC[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO Channel 03 : 2[190] -> 1[180] via P2P/IPC[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO Channel 05 : 2[190] -> 6[1d0] via P2P/IPC[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO Channel 05 : 4[1b0] -> 5[1c0] via P2P/IPC[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO Channel 04 : 3[1a0] -> 7[1e0] via P2P/IPC[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO Channel 05 : 3[1a0] -> 1[180] via P2P/IPC[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Channel 05 : 0[170] -> 2[190] via P2P/IPC[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO Channel 05 : 5[1c0] -> 7[1e0] via P2P/IPC[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO Channel 05 : 6[1d0] -> 4[1b0] via P2P/IPC[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO Channel 04 : 0[170] -> 1[180] via P2P/IPC[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO Channel 05 : 7[1e0] -> 3[1a0] via P2P/IPC[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO Channel 04 : 4[1b0] -> 6[1d0] via P2P/IPC[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO Channel 05 : 1[180] -> 0[170] via P2P/IPC[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO Channel 04 : 5[1c0] -> 4[1b0] via P2P/IPC[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO Channel 04 : 6[1d0] -> 2[190] via P2P/IPC[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO Channel 04 : 7[1e0] -> 5[1c0] via P2P/IPC[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO Channel 04 : 1[180] -> 3[1a0] via P2P/IPC[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO Channel 04 : 2[190] -> 0[170] via P2P/IPC[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO Channel 05 : 2[190] -> 0[170] via P2P/IPC[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO Channel 05 : 1[180] -> 3[1a0] via P2P/IPC[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO Channel 05 : 4[1b0] -> 6[1d0] via P2P/IPC[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO Channel 05 : 3[1a0] -> 7[1e0] via P2P/IPC[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO Channel 05 : 5[1c0] -> 4[1b0] via P2P/IPC[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO Channel 05 : 6[1d0] -> 2[190] via P2P/IPC[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO Channel 05 : 7[1e0] -> 5[1c0] via P2P/IPC[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO Channel 04 : 2[190] -> 6[1d0] via P2P/IPC[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO Channel 04 : 3[1a0] -> 1[180] via P2P/IPC[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO Channel 04 : 4[1b0] -> 5[1c0] via P2P/IPC[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Channel 06 : 0[170] -> 3[1a0] via P2P/IPC[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO Channel 06 : 1[180] -> 5[1c0] via P2P/IPC[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO Channel 06 : 2[190] -> 1[180] via P2P/IPC[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO Channel 06 : 4[1b0] -> 0[170] via P2P/IPC[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO Channel 06 : 3[1a0] -> 2[190] via P2P/IPC[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO Channel 06 : 5[1c0] -> 6[1d0] via P2P/IPC[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO Channel 06 : 6[1d0] -> 7[1e0] via P2P/IPC[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO Channel 06 : 7[1e0] -> 4[1b0] via P2P/IPC[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO Channel 04 : 5[1c0] -> 7[1e0] via P2P/IPC[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO Channel 04 : 6[1d0] -> 4[1b0] via P2P/IPC[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO Channel 04 : 7[1e0] -> 3[1a0] via P2P/IPC[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO Channel 04 : 1[180] -> 0[170] via P2P/IPC[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO Channel 06 : 4[1b0] -> 7[1e0] via P2P/IPC[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO Channel 06 : 1[180] -> 2[190] via P2P/IPC[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO Channel 06 : 2[190] -> 3[1a0] via P2P/IPC[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO Channel 06 : 3[1a0] -> 0[170] via P2P/IPC[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO Channel 06 : 5[1c0] -> 1[180] via P2P/IPC[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO Channel 05 : 2[190] -> 6[1d0] via P2P/IPC[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO Channel 06 : 6[1d0] -> 5[1c0] via P2P/IPC[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO Channel 06 : 7[1e0] -> 6[1d0] via P2P/IPC[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO Channel 05 : 3[1a0] -> 1[180] via P2P/IPC[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO Channel 05 : 4[1b0] -> 5[1c0] via P2P/IPC[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO Channel 05 : 0[170] -> 2[190] via P2P/IPC[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO Channel 05 : 5[1c0] -> 7[1e0] via P2P/IPC[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO Channel 05 : 6[1d0] -> 4[1b0] via P2P/IPC[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO Channel 05 : 7[1e0] -> 3[1a0] via P2P/IPC[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO Channel 05 : 1[180] -> 0[170] via P2P/IPC[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO Channel 07 : 4[1b0] -> 0[170] via P2P/IPC[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Channel 07 : 0[170] -> 3[1a0] via P2P/IPC[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO Channel 07 : 1[180] -> 5[1c0] via P2P/IPC[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO Channel 07 : 2[190] -> 1[180] via P2P/IPC[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO Channel 07 : 3[1a0] -> 2[190] via P2P/IPC[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO Channel 07 : 5[1c0] -> 6[1d0] via P2P/IPC[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO Channel 07 : 6[1d0] -> 7[1e0] via P2P/IPC[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO Channel 07 : 7[1e0] -> 4[1b0] via P2P/IPC[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO Channel 05 : 2[190] -> 0[170] via P2P/IPC[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO Channel 05 : 1[180] -> 3[1a0] via P2P/IPC[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO Channel 05 : 3[1a0] -> 7[1e0] via P2P/IPC[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO Channel 05 : 4[1b0] -> 6[1d0] via P2P/IPC[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO Channel 05 : 6[1d0] -> 2[190] via P2P/IPC[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO Channel 05 : 5[1c0] -> 4[1b0] via P2P/IPC[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO Channel 05 : 7[1e0] -> 5[1c0] via P2P/IPC[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO Channel 07 : 4[1b0] -> 7[1e0] via P2P/IPC[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO Channel 07 : 1[180] -> 2[190] via P2P/IPC[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO Channel 07 : 2[190] -> 3[1a0] via P2P/IPC[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO Channel 07 : 3[1a0] -> 0[170] via P2P/IPC[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO Channel 07 : 5[1c0] -> 1[180] via P2P/IPC[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO Channel 07 : 6[1d0] -> 5[1c0] via P2P/IPC[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO Channel 07 : 7[1e0] -> 6[1d0] via P2P/IPC[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO Channel 06 : 0[170] -> 3[1a0] via P2P/IPC[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO Channel 06 : 1[180] -> 5[1c0] via P2P/IPC[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO Channel 06 : 2[190] -> 1[180] via P2P/IPC[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO Channel 06 : 3[1a0] -> 2[190] via P2P/IPC[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO Channel 06 : 4[1b0] -> 0[170] via P2P/IPC[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO Channel 06 : 6[1d0] -> 7[1e0] via P2P/IPC[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO Channel 06 : 5[1c0] -> 6[1d0] via P2P/IPC[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO Channel 06 : 7[1e0] -> 4[1b0] via P2P/IPC[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO Channel 08 : 4[1b0] -> 7[1e0] via P2P/IPC[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Channel 08 : 0[170] -> 4[1b0] via P2P/IPC[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO Channel 08 : 1[180] -> 2[190] via P2P/IPC[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO Channel 08 : 2[190] -> 3[1a0] via P2P/IPC[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO Channel 08 : 3[1a0] -> 0[170] via P2P/IPC[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO Channel 08 : 5[1c0] -> 1[180] via P2P/IPC[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO Channel 08 : 6[1d0] -> 5[1c0] via P2P/IPC[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO Channel 08 : 7[1e0] -> 6[1d0] via P2P/IPC[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO Channel 06 : 4[1b0] -> 7[1e0] via P2P/IPC[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO Channel 06 : 1[180] -> 2[190] via P2P/IPC[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO Channel 06 : 2[190] -> 3[1a0] via P2P/IPC[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO Channel 06 : 3[1a0] -> 0[170] via P2P/IPC[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO Channel 06 : 6[1d0] -> 5[1c0] via P2P/IPC[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO Channel 06 : 5[1c0] -> 1[180] via P2P/IPC[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO Channel 06 : 7[1e0] -> 6[1d0] via P2P/IPC[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO Channel 08 : 3[1a0] -> 2[190] via P2P/IPC[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO Channel 08 : 4[1b0] -> 0[170] via P2P/IPC[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO Channel 08 : 1[180] -> 5[1c0] via P2P/IPC[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO Channel 08 : 2[190] -> 1[180] via P2P/IPC[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO Channel 08 : 5[1c0] -> 6[1d0] via P2P/IPC[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO Channel 08 : 6[1d0] -> 7[1e0] via P2P/IPC[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO Channel 08 : 7[1e0] -> 4[1b0] via P2P/IPC[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO Channel 07 : 4[1b0] -> 0[170] via P2P/IPC[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO Channel 07 : 0[170] -> 3[1a0] via P2P/IPC[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO Channel 07 : 2[190] -> 1[180] via P2P/IPC[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO Channel 07 : 1[180] -> 5[1c0] via P2P/IPC[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO Channel 07 : 3[1a0] -> 2[190] via P2P/IPC[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO Channel 07 : 6[1d0] -> 7[1e0] via P2P/IPC[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO Channel 07 : 5[1c0] -> 6[1d0] via P2P/IPC[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO Channel 09 : 3[1a0] -> 0[170] via P2P/IPC[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO Channel 07 : 7[1e0] -> 4[1b0] via P2P/IPC[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Channel 09 : 0[170] -> 4[1b0] via P2P/IPC[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO Channel 09 : 1[180] -> 2[190] via P2P/IPC[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO Channel 09 : 2[190] -> 3[1a0] via P2P/IPC[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO Channel 09 : 4[1b0] -> 7[1e0] via P2P/IPC[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO Channel 09 : 5[1c0] -> 1[180] via P2P/IPC[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO Channel 09 : 6[1d0] -> 5[1c0] via P2P/IPC[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO Channel 07 : 4[1b0] -> 7[1e0] via P2P/IPC[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO Channel 09 : 7[1e0] -> 6[1d0] via P2P/IPC[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO Channel 07 : 2[190] -> 3[1a0] via P2P/IPC[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO Channel 07 : 1[180] -> 2[190] via P2P/IPC[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO Channel 07 : 3[1a0] -> 0[170] via P2P/IPC[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO Channel 09 : 3[1a0] -> 2[190] via P2P/IPC[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO Channel 07 : 6[1d0] -> 5[1c0] via P2P/IPC[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO Channel 07 : 5[1c0] -> 1[180] via P2P/IPC[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO Channel 07 : 7[1e0] -> 6[1d0] via P2P/IPC[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO Channel 09 : 2[190] -> 1[180] via P2P/IPC[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO Channel 09 : 1[180] -> 5[1c0] via P2P/IPC[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO Channel 09 : 4[1b0] -> 0[170] via P2P/IPC[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO Channel 09 : 5[1c0] -> 6[1d0] via P2P/IPC[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO Channel 09 : 6[1d0] -> 7[1e0] via P2P/IPC[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO Channel 09 : 7[1e0] -> 4[1b0] via P2P/IPC[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO Channel 08 : 4[1b0] -> 7[1e0] via P2P/IPC[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO Channel 08 : 0[170] -> 4[1b0] via P2P/IPC[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO Channel 08 : 2[190] -> 3[1a0] via P2P/IPC[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO Channel 08 : 1[180] -> 2[190] via P2P/IPC[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO Channel 10 : 3[1a0] -> 7[1e0] via P2P/IPC[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO Channel 08 : 3[1a0] -> 0[170] via P2P/IPC[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO Channel 08 : 6[1d0] -> 5[1c0] via P2P/IPC[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO Channel 08 : 5[1c0] -> 1[180] via P2P/IPC[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO Channel 08 : 7[1e0] -> 6[1d0] via P2P/IPC[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Channel 10 : 0[170] -> 1[180] via P2P/IPC[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO Channel 10 : 1[180] -> 3[1a0] via P2P/IPC[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO Channel 10 : 2[190] -> 0[170] via P2P/IPC[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO Channel 10 : 4[1b0] -> 6[1d0] via P2P/IPC[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO Channel 10 : 5[1c0] -> 4[1b0] via P2P/IPC[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO Channel 10 : 6[1d0] -> 2[190] via P2P/IPC[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO Channel 10 : 7[1e0] -> 5[1c0] via P2P/IPC[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO Channel 08 : 3[1a0] -> 2[190] via P2P/IPC[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO Channel 08 : 2[190] -> 1[180] via P2P/IPC[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO Channel 08 : 4[1b0] -> 0[170] via P2P/IPC[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO Channel 08 : 1[180] -> 5[1c0] via P2P/IPC[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO Channel 08 : 6[1d0] -> 7[1e0] via P2P/IPC[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO Channel 08 : 5[1c0] -> 6[1d0] via P2P/IPC[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO Channel 10 : 2[190] -> 6[1d0] via P2P/IPC[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO Channel 08 : 7[1e0] -> 4[1b0] via P2P/IPC[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO Channel 10 : 3[1a0] -> 1[180] via P2P/IPC[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO Channel 10 : 1[180] -> 0[170] via P2P/IPC[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO Channel 10 : 4[1b0] -> 5[1c0] via P2P/IPC[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO Channel 10 : 5[1c0] -> 7[1e0] via P2P/IPC[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO Channel 09 : 3[1a0] -> 0[170] via P2P/IPC[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO Channel 10 : 6[1d0] -> 4[1b0] via P2P/IPC[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO Channel 10 : 7[1e0] -> 3[1a0] via P2P/IPC[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO Channel 09 : 0[170] -> 4[1b0] via P2P/IPC[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO Channel 09 : 2[190] -> 3[1a0] via P2P/IPC[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO Channel 09 : 1[180] -> 2[190] via P2P/IPC[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO Channel 09 : 4[1b0] -> 7[1e0] via P2P/IPC[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO Channel 09 : 6[1d0] -> 5[1c0] via P2P/IPC[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO Channel 09 : 5[1c0] -> 1[180] via P2P/IPC[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO Channel 11 : 2[190] -> 6[1d0] via P2P/IPC[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO Channel 09 : 7[1e0] -> 6[1d0] via P2P/IPC[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Channel 11 : 0[170] -> 2[190] via P2P/IPC[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO Channel 11 : 1[180] -> 0[170] via P2P/IPC[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO Channel 09 : 3[1a0] -> 2[190] via P2P/IPC[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO Channel 11 : 4[1b0] -> 5[1c0] via P2P/IPC[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO Channel 11 : 3[1a0] -> 1[180] via P2P/IPC[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO Channel 11 : 5[1c0] -> 7[1e0] via P2P/IPC[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO Channel 11 : 6[1d0] -> 4[1b0] via P2P/IPC[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO Channel 11 : 7[1e0] -> 3[1a0] via P2P/IPC[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO Channel 09 : 2[190] -> 1[180] via P2P/IPC[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO Channel 09 : 1[180] -> 5[1c0] via P2P/IPC[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO Channel 11 : 1[180] -> 3[1a0] via P2P/IPC[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO Channel 09 : 4[1b0] -> 0[170] via P2P/IPC[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO Channel 09 : 6[1d0] -> 7[1e0] via P2P/IPC[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO Channel 09 : 5[1c0] -> 6[1d0] via P2P/IPC[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO Channel 11 : 2[190] -> 0[170] via P2P/IPC[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO Channel 09 : 7[1e0] -> 4[1b0] via P2P/IPC[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO Channel 11 : 4[1b0] -> 6[1d0] via P2P/IPC[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO Channel 11 : 3[1a0] -> 7[1e0] via P2P/IPC[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO Channel 11 : 5[1c0] -> 4[1b0] via P2P/IPC[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO Channel 11 : 6[1d0] -> 2[190] via P2P/IPC[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO Channel 10 : 3[1a0] -> 7[1e0] via P2P/IPC[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO Channel 11 : 7[1e0] -> 5[1c0] via P2P/IPC[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO 12 coll channels, 16 p2p channels, 2 p2p channels per peer[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO comm 0x55e12a1f8d20 rank 1 nranks 8 cudaDev 1 busId 180 - Init COMPLETE[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO 12 coll channels, 16 p2p channels, 2 p2p channels per peer[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO comm 0x55e0044da6f0 rank 0 nranks 8 cudaDev 0 busId 170 - Init COMPLETE[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO 12 coll channels, 16 p2p channels, 2 p2p channels per peer[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO comm 0x55d36e091780 rank 2 nranks 8 cudaDev 2 busId 190 - Init COMPLETE[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO 12 coll channels, 16 p2p channels, 2 p2p channels per peer[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO 12 coll channels, 16 p2p channels, 2 p2p channels per peer[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO comm 0x55e101b84f90 rank 4 nranks 8 cudaDev 4 busId 1b0 - Init COMPLETE[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO Channel 10 : 0[170] -> 1[180] via P2P/IPC[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO Channel 10 : 2[190] -> 0[170] via P2P/IPC[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO comm 0x559f01970770 rank 3 nranks 8 cudaDev 3 busId 1a0 - Init COMPLETE[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO 12 coll channels, 16 p2p channels, 2 p2p channels per peer[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO 12 coll channels, 16 p2p channels, 2 p2p channels per peer[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO comm 0x5649b8f6dbf0 rank 5 nranks 8 cudaDev 5 busId 1c0 - Init COMPLETE[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO comm 0x55d690cb2d90 rank 6 nranks 8 cudaDev 6 busId 1d0 - Init COMPLETE[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO 12 coll channels, 16 p2p channels, 2 p2p channels per peer[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO Channel 10 : 1[180] -> 3[1a0] via P2P/IPC[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO comm 0x5558a63eacc0 rank 7 nranks 8 cudaDev 7 busId 1e0 - Init COMPLETE[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO Channel 10 : 4[1b0] -> 6[1d0] via P2P/IPC[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO Channel 10 : 5[1c0] -> 4[1b0] via P2P/IPC[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO Channel 10 : 6[1d0] -> 2[190] via P2P/IPC[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO Channel 10 : 7[1e0] -> 5[1c0] via P2P/IPC[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO Channel 10 : 2[190] -> 6[1d0] via P2P/IPC[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO Channel 10 : 3[1a0] -> 1[180] via P2P/IPC[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO Channel 10 : 1[180] -> 0[170] via P2P/IPC[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO Channel 10 : 4[1b0] -> 5[1c0] via P2P/IPC[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO Channel 10 : 6[1d0] -> 4[1b0] via P2P/IPC[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO Channel 10 : 5[1c0] -> 7[1e0] via P2P/IPC[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO Channel 10 : 7[1e0] -> 3[1a0] via P2P/IPC[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO Channel 11 : 2[190] -> 6[1d0] via P2P/IPC[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO Channel 11 : 0[170] -> 2[190] via P2P/IPC[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO Channel 11 : 1[180] -> 0[170] via P2P/IPC[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO Channel 11 : 3[1a0] -> 1[180] via P2P/IPC[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO Channel 11 : 4[1b0] -> 5[1c0] via P2P/IPC[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO Channel 11 : 6[1d0] -> 4[1b0] via P2P/IPC[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO Channel 11 : 5[1c0] -> 7[1e0] via P2P/IPC[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO Channel 11 : 7[1e0] -> 3[1a0] via P2P/IPC[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO Channel 11 : 1[180] -> 3[1a0] via P2P/IPC[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO Channel 11 : 2[190] -> 0[170] via P2P/IPC[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO Channel 11 : 3[1a0] -> 7[1e0] via P2P/IPC[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO Channel 11 : 4[1b0] -> 6[1d0] via P2P/IPC[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO Channel 11 : 5[1c0] -> 4[1b0] via P2P/IPC[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO Channel 11 : 6[1d0] -> 2[190] via P2P/IPC[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO 12 coll channels, 16 p2p channels, 2 p2p channels per peer[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO Channel 11 : 7[1e0] -> 5[1c0] via P2P/IPC[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO comm 0x55bd068d5e00 rank 1 nranks 8 cudaDev 1 busId 180 - Init COMPLETE[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO 12 coll channels, 16 p2p channels, 2 p2p channels per peer[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO comm 0x56329979e5c0 rank 0 nranks 8 cudaDev 0 busId 170 - Init COMPLETE[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO 12 coll channels, 16 p2p channels, 2 p2p channels per peer[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO 12 coll channels, 16 p2p channels, 2 p2p channels per peer[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO comm 0x5569a2622ce0 rank 2 nranks 8 cudaDev 2 busId 190 - Init COMPLETE[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO 12 coll channels, 16 p2p channels, 2 p2p channels per peer[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO comm 0x55e53a2130b0 rank 3 nranks 8 cudaDev 3 busId 1a0 - Init COMPLETE[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO comm 0x5589379f74c0 rank 4 nranks 8 cudaDev 4 busId 1b0 - Init COMPLETE[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO 12 coll channels, 16 p2p channels, 2 p2p channels per peer[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO comm 0x55eb4a4d0050 rank 6 nranks 8 cudaDev 6 busId 1d0 - Init COMPLETE[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO 12 coll channels, 16 p2p channels, 2 p2p channels per peer[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO 12 coll channels, 16 p2p channels, 2 p2p channels per peer[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO comm 0x55989e69e640 rank 5 nranks 8 cudaDev 5 busId 1c0 - Init COMPLETE[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO comm 0x55e1486a78e0 rank 7 nranks 8 cudaDev 7 busId 1e0 - Init COMPLETE[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO threadThresholds 8/8/64 | 128/8/64 | 8/8/64[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO Trees [0] -1/-1/-1->4->7|7->4->-1/-1/-1 [1] -1/-1/-1->4->7|7->4->-1/-1/-1[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO threadThresholds 8/8/64 | 128/8/64 | 8/8/64[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO Trees [0] 2/8/-1->3->0|0->3->2/8/-1 [1] 2/-1/-1->3->0|0->3->2/-1/-1[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO threadThresholds 8/8/64 | 128/8/64 | 8/8/64[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO Trees [0] 6/-1/-1->5->1|1->5->6/-1/-1 [1] 6/-1/-1->5->1|1->5->6/-1/-1[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO threadThresholds 8/8/64 | 128/8/64 | 8/8/64[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO Trees [0] 5/-1/-1->1->2|2->1->5/-1/-1 [1] 5/-1/-1->1->2|2->1->5/-1/-1[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO threadThresholds 8/8/64 | 128/8/64 | 8/8/64[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO Trees [0] 1/-1/-1->2->3|3->2->1/-1/-1 [1] 1/-1/-1->2->3|3->2->1/-1/-1[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Channel 00/02 : 0 3 2 1 5 6 7 4 8 11 10 9 13 14 15 12[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Channel 01/02 : 0 3 2 1 5 6 7 4 8 11 10 9 13 14 15 12[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO threadThresholds 8/8/64 | 128/8/64 | 8/8/64[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Trees [0] 3/-1/-1->0->-1|-1->0->3/-1/-1 [1] 3/-1/-1->0->11|11->0->3/-1/-1[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO threadThresholds 8/8/64 | 128/8/64 | 8/8/64[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO Trees [0] 4/-1/-1->7->6|6->7->4/-1/-1 [1] 4/-1/-1->7->6|6->7->4/-1/-1[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO threadThresholds 8/8/64 | 128/8/64 | 8/8/64[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO Trees [0] 7/-1/-1->6->5|5->6->7/-1/-1 [1] 7/-1/-1->6->5|5->6->7/-1/-1[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO threadThresholds 8/8/64 | 128/8/64 | 8/8/64[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO Trees [0] 12/-1/-1->15->14|14->15->12/-1/-1 [1] 12/-1/-1->15->14|14->15->12/-1/-1[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO threadThresholds 8/8/64 | 128/8/64 | 8/8/64[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO Trees [0] -1/-1/-1->12->15|15->12->-1/-1/-1 [1] -1/-1/-1->12->15|15->12->-1/-1/-1[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO threadThresholds 8/8/64 | 128/8/64 | 8/8/64[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO Trees [0] 14/-1/-1->13->9|9->13->14/-1/-1 [1] 14/-1/-1->13->9|9->13->14/-1/-1[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO threadThresholds 8/8/64 | 128/8/64 | 8/8/64[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO Trees [0] 15/-1/-1->14->13|13->14->15/-1/-1 [1] 15/-1/-1->14->13|13->14->15/-1/-1[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO threadThresholds 8/8/64 | 128/8/64 | 8/8/64[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO Trees [0] 11/-1/-1->8->3|3->8->11/-1/-1 [1] 11/-1/-1->8->-1|-1->8->11/-1/-1[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO threadThresholds 8/8/64 | 128/8/64 | 8/8/64[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO Trees [0] 13/-1/-1->9->10|10->9->13/-1/-1 [1] 13/-1/-1->9->10|10->9->13/-1/-1[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO threadThresholds 8/8/64 | 128/8/64 | 8/8/64[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO threadThresholds 8/8/64 | 128/8/64 | 8/8/64[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO Trees [0] 10/-1/-1->11->8|8->11->10/-1/-1 [1] 10/0/-1->11->8|8->11->10/0/-1[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO Trees [0] 9/-1/-1->10->11|11->10->9/-1/-1 [1] 9/-1/-1->10->11|11->10->9/-1/-1[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO Channel 00 : 15[1e0] -> 12[1b0] via P2P/IPC[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO Channel 00 : 2[190] -> 1[180] via P2P/IPC[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO Channel 00 : 13[1c0] -> 14[1d0] via P2P/IPC[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO Channel 00 : 14[1d0] -> 15[1e0] via P2P/IPC[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO Channel 00 : 1[180] -> 5[1c0] via P2P/IPC[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO Channel 00 : 3[1a0] -> 2[190] via P2P/IPC[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO Channel 00 : 6[1d0] -> 7[1e0] via P2P/IPC[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO Channel 00 : 5[1c0] -> 6[1d0] via P2P/IPC[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO Channel 00 : 7[1e0] -> 4[1b0] via P2P/IPC[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO Channel 00 : 9[180] -> 13[1c0] via P2P/IPC[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO Channel 00 : 11[1a0] -> 10[190] via P2P/IPC[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO Channel 00 : 10[190] -> 9[180] via P2P/IPC[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Channel 00 : 12[1b0] -> 0[170] [receive] via NET/Socket/0[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO NET/Socket: Using 2 threads and 8 sockets per thread[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO Channel 00 : 4[1b0] -> 8[170] [receive] via NET/Socket/0[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO NET/Socket: Using 2 threads and 8 sockets per thread[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO Channel 00 : 4[1b0] -> 8[170] [send] via NET/Socket/0[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO Channel 00 : 12[1b0] -> 0[170] [send] via NET/Socket/0[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO Channel 00 : 8[170] -> 11[1a0] via P2P/IPC[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Channel 00 : 0[170] -> 3[1a0] via P2P/IPC[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO Channel 00 : 15[1e0] -> 14[1d0] via P2P/IPC[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO Channel 00 : 2[190] -> 3[1a0] via P2P/IPC[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO Channel 00 : 1[180] -> 2[190] via P2P/IPC[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO Channel 00 : 13[1c0] -> 9[180] via P2P/IPC[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO Channel 00 : 14[1d0] -> 13[1c0] via P2P/IPC[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO Channel 00 : 5[1c0] -> 1[180] via P2P/IPC[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO Channel 00 : 6[1d0] -> 5[1c0] via P2P/IPC[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO Channel 00 : 7[1e0] -> 6[1d0] via P2P/IPC[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO Channel 00 : 9[180] -> 10[190] via P2P/IPC[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO Channel 00 : 10[190] -> 11[1a0] via P2P/IPC[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO Channel 00 : 11[1a0] -> 8[170] via P2P/IPC[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO Channel 00 : 12[1b0] -> 15[1e0] via P2P/IPC[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO Channel 00 : 4[1b0] -> 7[1e0] via P2P/IPC[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO Channel 00 : 8[170] -> 3[1a0] [receive] via NET/Socket/0[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO NET/Socket: Using 2 threads and 8 sockets per thread[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO Channel 00 : 8[170] -> 3[1a0] [send] via NET/Socket/0[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO Channel 01 : 1[180] -> 5[1c0] via P2P/IPC[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO Channel 00 : 3[1a0] -> 0[170] via P2P/IPC[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO Channel 01 : 2[190] -> 1[180] via P2P/IPC[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO Channel 01 : 6[1d0] -> 7[1e0] via P2P/IPC[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO Channel 01 : 5[1c0] -> 6[1d0] via P2P/IPC[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO Channel 01 : 13[1c0] -> 14[1d0] via P2P/IPC[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO Channel 01 : 14[1d0] -> 15[1e0] via P2P/IPC[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO Channel 01 : 9[180] -> 13[1c0] via P2P/IPC[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO Channel 01 : 10[190] -> 9[180] via P2P/IPC[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO Channel 01 : 7[1e0] -> 4[1b0] via P2P/IPC[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO Channel 01 : 15[1e0] -> 12[1b0] via P2P/IPC[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO Channel 01 : 11[1a0] -> 10[190] via P2P/IPC[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO Channel 01 : 4[1b0] -> 8[170] [send] via NET/Socket/0[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO Channel 01 : 12[1b0] -> 0[170] [send] via NET/Socket/0[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Channel 01 : 12[1b0] -> 0[170] [receive] via NET/Socket/0[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO NET/Socket: Using 2 threads and 8 sockets per thread[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO Channel 01 : 1[180] -> 2[190] via P2P/IPC[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO Channel 01 : 6[1d0] -> 5[1c0] via P2P/IPC[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO Channel 01 : 5[1c0] -> 1[180] via P2P/IPC[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO Channel 01 : 7[1e0] -> 6[1d0] via P2P/IPC[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO Channel 01 : 14[1d0] -> 13[1c0] via P2P/IPC[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO Channel 01 : 13[1c0] -> 9[180] via P2P/IPC[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Channel 01 : 0[170] -> 3[1a0] via P2P/IPC[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO Channel 01 : 9[180] -> 10[190] via P2P/IPC[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO Channel 00 : 3[1a0] -> 8[170] [send] via NET/Socket/0[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO Channel 01 : 10[190] -> 11[1a0] via P2P/IPC[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO Channel 01 : 15[1e0] -> 14[1d0] via P2P/IPC[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO Channel 00 : 3[1a0] -> 8[170] [receive] via NET/Socket/0[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO NET/Socket: Using 2 threads and 8 sockets per thread[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO 2 coll channels, 2 p2p channels, 1 p2p channels per peer[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO 2 coll channels, 2 p2p channels, 1 p2p channels per peer[0m
[34m[1,6]<stdout>:algo-1:214:214 [6] NCCL INFO comm 0x55d693988f70 rank 6 nranks 16 cudaDev 6 busId 1d0 - Init COMPLETE[0m
[34m[1,5]<stdout>:algo-1:216:216 [5] NCCL INFO comm 0x5649bbc43dd0 rank 5 nranks 16 cudaDev 5 busId 1c0 - Init COMPLETE[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO 2 coll channels, 2 p2p channels, 1 p2p channels per peer[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO 2 coll channels, 2 p2p channels, 1 p2p channels per peer[0m
[34m[1,13]<stdout>:algo-2:229:229 [5] NCCL INFO comm 0x5598a1374820 rank 13 nranks 16 cudaDev 5 busId 1c0 - Init COMPLETE[0m
[34m[1,14]<stdout>:algo-2:227:227 [6] NCCL INFO comm 0x55eb4d1a6230 rank 14 nranks 16 cudaDev 6 busId 1d0 - Init COMPLETE[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO 2 coll channels, 2 p2p channels, 1 p2p channels per peer[0m
[34m[1,9]<stdout>:algo-2:223:223 [1] NCCL INFO comm 0x55bd095abfe0 rank 9 nranks 16 cudaDev 1 busId 180 - Init COMPLETE[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO Channel 01 : 12[1b0] -> 15[1e0] via P2P/IPC[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO 2 coll channels, 2 p2p channels, 1 p2p channels per peer[0m
[34m[1,15]<stdout>:algo-2:226:226 [7] NCCL INFO comm 0x55e14b37dac0 rank 15 nranks 16 cudaDev 7 busId 1e0 - Init COMPLETE[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO 2 coll channels, 2 p2p channels, 1 p2p channels per peer[0m
[34m[1,12]<stdout>:algo-2:225:225 [4] NCCL INFO comm 0x55893a6cd6a0 rank 12 nranks 16 cudaDev 4 busId 1b0 - Init COMPLETE[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO Channel 01 : 3[1a0] -> 2[190] via P2P/IPC[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO Channel 01 : 2[190] -> 3[1a0] via P2P/IPC[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO Channel 01 : 3[1a0] -> 0[170] via P2P/IPC[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO 2 coll channels, 2 p2p channels, 1 p2p channels per peer[0m
[34m[1,1]<stdout>:algo-1:219:219 [1] NCCL INFO comm 0x55e12cecef00 rank 1 nranks 16 cudaDev 1 busId 180 - Init COMPLETE[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO Channel 01 : 4[1b0] -> 8[170] [receive] via NET/Socket/0[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO NET/Socket: Using 2 threads and 8 sockets per thread[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO 2 coll channels, 2 p2p channels, 1 p2p channels per peer[0m
[34m[1,2]<stdout>:algo-1:217:217 [2] NCCL INFO comm 0x55d370d67960 rank 2 nranks 16 cudaDev 2 busId 190 - Init COMPLETE[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO Channel 01 : 8[170] -> 11[1a0] via P2P/IPC[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO 2 coll channels, 2 p2p channels, 1 p2p channels per peer[0m
[34m[1,3]<stdout>:algo-1:218:218 [3] NCCL INFO comm 0x559f04646950 rank 3 nranks 16 cudaDev 3 busId 1a0 - Init COMPLETE[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Channel 01 : 0[170] -> 11[1a0] [send] via NET/Socket/0[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO 2 coll channels, 2 p2p channels, 1 p2p channels per peer[0m
[34m[1,10]<stdout>:algo-2:228:228 [2] NCCL INFO comm 0x5569a52f8ec0 rank 10 nranks 16 cudaDev 2 busId 190 - Init COMPLETE[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO Channel 01 : 4[1b0] -> 7[1e0] via P2P/IPC[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO 2 coll channels, 2 p2p channels, 1 p2p channels per peer[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO 2 coll channels, 2 p2p channels, 1 p2p channels per peer[0m
[34m[1,7]<stdout>:algo-1:220:220 [7] NCCL INFO comm 0x5558a90c0ea0 rank 7 nranks 16 cudaDev 7 busId 1e0 - Init COMPLETE[0m
[34m[1,4]<stdout>:algo-1:215:215 [4] NCCL INFO comm 0x55e10485b170 rank 4 nranks 16 cudaDev 4 busId 1b0 - Init COMPLETE[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO Channel 01 : 0[170] -> 11[1a0] [receive] via NET/Socket/0[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO NET/Socket: Using 2 threads and 8 sockets per thread[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO Channel 01 : 11[1a0] -> 8[170] via P2P/IPC[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO 2 coll channels, 2 p2p channels, 1 p2p channels per peer[0m
[34m[1,8]<stdout>:algo-2:678:678 [0] NCCL INFO comm 0x56329c4747a0 rank 8 nranks 16 cudaDev 0 busId 170 - Init COMPLETE[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO Channel 01 : 11[1a0] -> 0[170] [send] via NET/Socket/0[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO Channel 01 : 11[1a0] -> 0[170] [receive] via NET/Socket/0[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO NET/Socket: Using 2 threads and 8 sockets per thread[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO 2 coll channels, 2 p2p channels, 1 p2p channels per peer[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO 2 coll channels, 2 p2p channels, 1 p2p channels per peer[0m
[34m[1,0]<stdout>:algo-1:669:669 [0] NCCL INFO comm 0x55e0071b08d0 rank 0 nranks 16 cudaDev 0 busId 170 - Init COMPLETE[0m
[34m[1,11]<stdout>:algo-2:224:224 [3] NCCL INFO comm 0x55e53cee9290 rank 11 nranks 16 cudaDev 3 busId 1a0 - Init COMPLETE[0m
[34m[1,0]<stdout>:Running smdistributed.dataparallel v1.2.0[0m
[34m[1,0]<stdout>:## dist.rank(): 0[0m
[34m[1,0]<stdout>:## args: [0m
[34m[1,0]<stdout>: Namespace(epochs=50, eval='/opt/ml/input/data/eval', eval_batch_size=512, learning_rate=6.25e-05, model_dir='s3://sagemaker-us-east-1-057716757052/cifar10-sm-ddp-2021-10-10-05-27-44-881/model', model_output_dir='/opt/ml/model', momentum=0.9, optimizer='adam', print_interval=100, train='/opt/ml/input/data/train', train_batch_size=64, validation='/opt/ml/input/data/validation', validation_batch_size=512, weight_decay=0.0002)[0m
[34m[1,8]<stdout>:[0m
[34m[1,8]<stdout>:################# Loading Dataset ################[0m
[34m[1,8]<stdout>:[0m
[34m[1,8]<stdout>:Channel Name: train[0m
[34m[1,8]<stdout>:[0m
[34m[1,8]<stdout>:# of batches loading TFRecord : 40000[0m
[34m[1,0]<stdout>:[0m
[34m[1,0]<stdout>:################# Loading Dataset ################[0m
[34m[1,0]<stdout>:[0m
[34m[1,0]<stdout>:Channel Name: train[0m
[34m[1,0]<stdout>:[0m
[34m[1,0]<stdout>:# of batches loading TFRecord : 40000[0m
[34m[1,8]<stdout>:buffer_size: 40000[0m
[34m[1,0]<stdout>:buffer_size: 40000[0m
[34m[1,8]<stdout>:[0m
[34m[1,8]<stdout>:################# Loading Dataset ################[0m
[34m[1,8]<stdout>:[0m
[34m[1,8]<stdout>:Channel Name: eval[0m
[34m[1,8]<stdout>:[0m
[34m[1,8]<stdout>:# of batches loading TFRecord : 10000[0m
[34m[1,0]<stdout>:[0m
[34m[1,0]<stdout>:################# Loading Dataset ################[0m
[34m[1,0]<stdout>:[0m
[34m[1,0]<stdout>:Channel Name: eval[0m
[34m[1,0]<stdout>:[0m
[34m[1,0]<stdout>:# of batches loading TFRecord : 10000[0m
[34m[1,10]<stdout>:[0m
[34m[1,10]<stdout>:################# Start Training ################[0m
[34m[1,13]<stdout>:[0m
[34m[1,13]<stdout>:################# Start Training ################[0m
[34m[1,10]<stdout>:## num_train_batch on each GPU10 : 39 [0m
[34m[1,8]<stdout>:[0m
[34m[1,8]<stdout>:################# Loading Dataset ################[0m
[34m[1,8]<stdout>:[0m
[34m[1,8]<stdout>:Channel Name: validation[0m
[34m[1,8]<stdout>:[0m
[34m[1,8]<stdout>:# of batches loading TFRecord : 10000[0m
[34m[1,13]<stdout>:## num_train_batch on each GPU13 : 39 [0m
[34m[1,14]<stdout>:[0m
[34m[1,14]<stdout>:################# Start Training ################[0m
[34m[1,14]<stdout>:## num_train_batch on each GPU14 : 39 [1,14]<stdout>:[0m
[34m[1,11]<stdout>:[0m
[34m[1,11]<stdout>:################# Start Training ################[0m
[34m[1,11]<stdout>:## num_train_batch on each GPU11 : 39 [0m
[34m[1,0]<stdout>:[0m
[34m[1,0]<stdout>:################# Loading Dataset ################[0m
[34m[1,0]<stdout>:[0m
[34m[1,0]<stdout>:Channel Name: validation[0m
[34m[1,0]<stdout>:[0m
[34m[1,0]<stdout>:# of batches loading TFRecord : 10000[0m
[34m[1,12]<stdout>:[0m
[34m[1,12]<stdout>:################# Start Training ################[1,12]<stdout>:[0m
[34m[1,12]<stdout>:## num_train_batch on each GPU12 : 39 [0m
[34m[1,8]<stdout>:[0m
[34m[1,8]<stdout>:################# Start Training ################[0m
[34m[1,8]<stdout>:## num_train_batch on each GPU8 : 39 [0m
[34m[1,9]<stdout>:[0m
[34m[1,9]<stdout>:################# Start Training ################[0m
[34m[1,9]<stdout>:## num_train_batch on each GPU9 : 39 [0m
[34m[1,15]<stdout>:[0m
[34m[1,15]<stdout>:################# Start Training ################[0m
[34m[1,15]<stdout>:## num_train_batch on each GPU15 : 39 [0m
[34m[1,2]<stdout>:[0m
[34m[1,2]<stdout>:################# Start Training ################[1,2]<stdout>:[0m
[34m[1,3]<stdout>:[0m
[34m[1,3]<stdout>:################# Start Training ################[1,3]<stdout>:[0m
[34m[1,2]<stdout>:## num_train_batch on each GPU2 : 39 [1,2]<stdout>:[0m
[34m[1,3]<stdout>:## num_train_batch on each GPU3 : 39 [0m
[34m[1,4]<stdout>:[0m
[34m[1,4]<stdout>:################# Start Training ################[1,4]<stdout>:[0m
[34m[1,4]<stdout>:## num_train_batch on each GPU4 : 39 [0m
[34m[1,1]<stdout>:[0m
[34m[1,1]<stdout>:################# Start Training ################[0m
[34m[1,6]<stdout>:[0m
[34m[1,6]<stdout>:################# Start Training ################[0m
[34m[1,1]<stdout>:## num_train_batch on each GPU1 : 39 [1,1]<stdout>:[0m
[34m[1,6]<stdout>:## num_train_batch on each GPU6 : 39 [0m
[34m[1,7]<stdout>:[0m
[34m[1,7]<stdout>:################# Start Training ################[1,7]<stdout>:[0m
[34m[1,7]<stdout>:## num_train_batch on each GPU7 : 39 [0m
[34m[1,0]<stdout>:[0m
[34m[1,0]<stdout>:################# Prepare Dataset ################[0m
[34m[1,0]<stdout>:# of batches in train: 625[0m
[34m[1,0]<stdout>:# of batches in eval: 19[0m
[34m[1,0]<stdout>:# of batches in validation: [1,0]<stdout>: 19[0m
[34m[1,0]<stdout>:[0m
[34m[1,0]<stdout>:################# Start Training ################[0m
[34m[1,5]<stdout>:[0m
[34m[1,5]<stdout>:################# Start Training ################[0m
[34m[1,0]<stdout>:## num_train_batch on each GPU0 : 39 [1,0]<stdout>:[0m
[34m[1,5]<stdout>:## num_train_batch on each GPU5 : 39 [1,5]<stdout>:[0m
[34m[1,0]<stdout>:algo-1:669:1465 [0] NCCL INFO Launch mode Parallel[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 45.839909[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 43.585342[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 46.942684[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 45.686176[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 44.264427[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 44.274006[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 42.006134[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 44.046497[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 43.531288[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 44.238415[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 46.214149[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 43.619919[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 44.220028[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 44.964233[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 44.133072[1,10]<stdout>:[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 45.316246[0m
[34m[1,0]<stdout>:algo-1:669:1457 [0] NCCL INFO Launch mode Parallel[0m
[34m[1,8]<stdout>:algo-2:678:1454 [0] NCCL INFO Launch mode Parallel[0m
[34m[1,0]<stdout>:## Epoch 1, Test Loss: 2.2932920455932617, Test Accuracy: 12.849506378173828[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 2.349646[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 2.307919[1,3]<stdout>:[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 2.360447[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 2.339609[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 2.308961[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 2.346674[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 2.346788[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 2.321728[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 2.330083[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 2.333593[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 2.347082[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 2.319762[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 2.349831[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 2.328220[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 2.313065[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 2.331002[0m
[34m[1,0]<stdout>:## Epoch 2, Test Loss: 2.228142261505127, Test Accuracy: 16.817434310913086[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 2.204259[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 2.210730[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 2.249399[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 2.241187[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 2.241482[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 2.172848[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 2.185448[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 2.198061[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 2.192755[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 2.157443[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 2.183794[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 2.195693[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 2.206168[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 2.226160[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 2.180345[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 2.173409[0m
[34m[1,0]<stdout>:## Epoch 3, Test Loss: 2.1646194458007812, Test Accuracy: 20.579771041870117[1,0]<stdout>:[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 2.086732[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 2.183975[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 2.220032[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 2.152664[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 2.245223[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 2.171533[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 2.240407[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 2.185227[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 2.120680[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 2.194559[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 2.149211[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 2.178373[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 2.150662[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 2.120291[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 2.219109[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 2.187619[0m
[34m[1,0]<stdout>:## Epoch 4, Test Loss: 2.089449882507324, Test Accuracy: 23.13939094543457[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.979374[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.969027[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.981454[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 2.006811[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 2.008089[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 2.032578[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 2.068012[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.986938[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 2.009119[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.975799[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 2.001479[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 2.037081[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 2.000236[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.974602[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.999527[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.983769[0m
[34m[1,0]<stdout>:## Epoch 5, Test Loss: 2.0608248710632324, Test Accuracy: 24.393503189086914[1,0]<stdout>:[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 2.136628[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 2.152581[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 2.084799[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 2.100378[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 2.119067[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 2.079105[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 2.085683[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 2.128334[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 2.162583[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 2.099816[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 2.095840[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 2.127154[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 2.128928[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 2.145758[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 2.155509[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 2.113157[0m
[34m[1,0]<stdout>:## Epoch 6, Test Loss: 2.0249857902526855, Test Accuracy: 25.390625[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 2.020013[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 2.050246[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 2.035357[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 2.005520[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.986283[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.926065[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 2.057175[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.982495[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.923061[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.897954[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.989905[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 2.046316[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.954777[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.990960[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 2.021283[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.977968[0m
[34m[1,0]<stdout>:## Epoch 7, Test Loss: 1.93934166431427, Test Accuracy: 29.31743621826172[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 2.035377[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 2.051547[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 2.081611[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.999087[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.991071[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 2.000966[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.983117[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.931333[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.933998[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.968261[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.923530[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.979462[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 2.029034[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 2.049215[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 2.034758[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.911745[0m
[34m[1,0]<stdout>:## Epoch 8, Test Loss: 1.9617747068405151, Test Accuracy: 29.00904655456543[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 2.192038[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 2.020018[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.955818[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.906562[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 2.001324[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 2.041116[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.993436[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.973218[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 2.028690[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.990092[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 2.035829[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.985818[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 2.118753[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 2.007382[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 2.041574[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 2.061645[0m
[34m[1,0]<stdout>:## Epoch 9, Test Loss: 1.9188705682754517, Test Accuracy: 30.160362243652344[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.839073[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.699414[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.765065[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.743294[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.822352[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.814817[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.831188[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.839593[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.857611[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.927654[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.800251[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.832536[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 2.014585[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.918193[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.868008[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.797777[0m
[34m[1,0]<stdout>:## Epoch 10, Test Loss: 1.858498215675354, Test Accuracy: 31.476152420043945[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.839853[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.899976[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.887146[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.871306[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.924761[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.840770[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.825081[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.855433[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.865525[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.895833[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.860290[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.853348[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.895833[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.884316[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.808072[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.731495[0m
[34m[1,0]<stdout>:## Epoch 11, Test Loss: 1.859017252922058, Test Accuracy: 32.689144134521484[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.903153[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.827846[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.963471[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.822337[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.929105[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.753518[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.885807[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.864776[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.904750[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 2.015070[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.943209[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.860258[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.952529[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.883009[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.852750[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.829074[0m
[34m[1,0]<stdout>:## Epoch 12, Test Loss: 1.7994033098220825, Test Accuracy: 35.76274871826172[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.764720[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.794784[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.649619[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.743676[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.664514[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.603223[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.728435[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.720337[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.734097[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.703719[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.716586[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.647323[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.682001[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.630172[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.695078[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.599853[0m
[34m[1,0]<stdout>:## Epoch 13, Test Loss: 1.7660819292068481, Test Accuracy: 35.690792083740234[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 2.083194[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.924928[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 2.035613[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.823593[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.926270[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.955554[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 2.062187[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.889693[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.897958[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.988777[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 2.050859[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.738231[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.916148[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.977754[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 2.016601[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 2.036379[0m
[34m[1,0]<stdout>:## Epoch 14, Test Loss: 1.743919014930725, Test Accuracy: 36.379520416259766[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.654151[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.668401[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.771017[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.669306[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.614620[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.756653[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.577072[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.651044[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.725126[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.554780[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.680734[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.765560[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.709464[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.596931[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.642842[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.678074[0m
[34m[1,0]<stdout>:## Epoch 15, Test Loss: 1.838932991027832, Test Accuracy: 32.79193878173828[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.764912[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.753032[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.833420[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.759365[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.743423[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.765882[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.748775[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.746893[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.907114[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.854281[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.800203[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.777554[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.789164[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.842387[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.678321[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.706113[0m
[34m[1,0]<stdout>:## Epoch 16, Test Loss: 1.7333890199661255, Test Accuracy: 36.55427551269531[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.933686[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.714017[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.779310[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.742447[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.775533[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.768480[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.663255[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.722139[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.728855[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.636390[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.790983[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.644175[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.821543[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.700338[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.727199[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.699447[0m
[34m[1,0]<stdout>:## Epoch 17, Test Loss: 1.6931126117706299, Test Accuracy: 38.0859375[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.516123[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.454476[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.607099[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.446403[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.595742[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.594234[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.491600[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.595417[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.553966[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.463848[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.536535[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.602677[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.629687[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.659824[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.592008[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.502763[0m
[34m[1,0]<stdout>:## Epoch 18, Test Loss: 1.702431082725525, Test Accuracy: 38.168174743652344[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.763875[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.759681[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.656237[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.726261[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.757860[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.806533[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.678478[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.791796[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.788912[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.661872[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.840188[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.706391[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.734707[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.838461[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.919004[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.628215[0m
[34m[1,0]<stdout>:## Epoch 19, Test Loss: 1.664872169494629, Test Accuracy: 38.83634948730469[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.378682[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.513130[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.529053[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.595858[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.472700[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.562994[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.474820[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.523322[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.522532[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.402438[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.484460[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.539697[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.481569[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.452791[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.550570[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.523510[0m
[34m[1,0]<stdout>:## Epoch 20, Test Loss: 1.6474651098251343, Test Accuracy: 40.86143112182617[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.639418[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.507773[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.701151[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.640999[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.595409[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.583609[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.667222[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.611266[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.671261[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.569252[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.627249[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.559892[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.586968[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.611916[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.622866[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.530730[0m
[34m[1,0]<stdout>:## Epoch 21, Test Loss: 1.6452316045761108, Test Accuracy: 40.32688903808594[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.501162[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.564316[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.509504[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.476982[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.614338[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.467690[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.468540[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.534021[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.506539[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.476847[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.397919[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.530908[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.515542[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.524400[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.471675[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.595164[0m
[34m[1,0]<stdout>:## Epoch 22, Test Loss: 1.6151189804077148, Test Accuracy: 41.40625[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.824179[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.652893[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.697019[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.650172[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.605875[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.651910[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.636697[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.681959[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.647024[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.801907[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.632272[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.523134[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.652245[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.559598[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.661298[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.631730[0m
[34m[1,0]<stdout>:## Epoch 23, Test Loss: 1.6220191717147827, Test Accuracy: 41.37541198730469[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.583097[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.554330[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.695420[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.506326[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.536209[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.608249[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.596654[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.727327[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.681723[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.677508[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.571900[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.570667[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.503957[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.691497[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.443841[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.510934[0m
[34m[1,0]<stdout>:## Epoch 24, Test Loss: 1.6810657978057861, Test Accuracy: 39.05221939086914[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.698009[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.713437[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.751998[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.709729[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.788019[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.713973[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.735436[0m
[34m[1,7]<stdout>:## GPU7 - Step #0[1,7]<stdout>:#011Loss: 1.614228[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.720342[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.681314[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.799915[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.657157[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.691873[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.709448[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.786941[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.865276[0m
[34m[1,0]<stdout>:## Epoch 25, Test Loss: 1.6059612035751343, Test Accuracy: 41.96134948730469[1,0]<stdout>:[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.528534[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.442390[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.521145[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.586349[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.561897[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.556373[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.542957[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.537731[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.516582[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.521762[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.590083[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.622888[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.622489[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.552043[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.605527[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.634891[0m
[34m[1,0]<stdout>:## Epoch 26, Test Loss: 1.634045124053955, Test Accuracy: 41.03618621826172[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.476300[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.479212[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.518364[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.645756[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.547462[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.622366[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.481725[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.498028[1,1]<stdout>:[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.514908[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.546532[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.490206[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.424710[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.621070[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.580447[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.449205[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.502324[0m
[34m[1,0]<stdout>:## Epoch 27, Test Loss: 1.5691734552383423, Test Accuracy: 43.68832015991211[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.495258[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.403007[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.363466[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.379307[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.363549[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.363935[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.508303[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.448810[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.361362[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.505590[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.299818[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.378384[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.435387[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.391885[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.386993[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.484010[0m
[34m[1,0]<stdout>:## Epoch 28, Test Loss: 1.7308467626571655, Test Accuracy: 38.89802551269531[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.839590[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.692863[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.795633[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.879225[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.825200[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.781097[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.844289[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.725756[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.777411[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.715868[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.778203[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.852152[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.915990[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.835864[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.863219[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.790404[0m
[34m[1,0]<stdout>:## Epoch 29, Test Loss: 1.5838629007339478, Test Accuracy: 43.15378189086914[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.519191[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.404632[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.574081[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.504246[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.458983[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.427045[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.519498[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.480248[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.450166[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.517814[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.506140[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.460701[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.525719[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.515195[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.395395[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.515061[0m
[34m[1,0]<stdout>:## Epoch 30, Test Loss: 1.5609078407287598, Test Accuracy: 43.29769515991211[1,0]<stdout>:[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.332935[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.497836[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.532038[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.418116[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.583804[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.462197[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.505162[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.560414[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.566807[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.524251[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.641262[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.641414[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.483527[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.516269[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.368886[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.605625[0m
[34m[1,0]<stdout>:## Epoch 31, Test Loss: 1.5532985925674438, Test Accuracy: 44.263980865478516[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.520648[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.471896[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.480098[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.555621[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.463971[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.657508[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.585021[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.588491[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.514520[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.550744[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.513976[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.544467[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.420960[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.422817[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.598803[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.481026[0m
[34m[1,0]<stdout>:## Epoch 32, Test Loss: 1.5801012516021729, Test Accuracy: 43.71916198730469[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.441072[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.378735[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.333895[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.527643[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.381052[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.451801[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.298435[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.467859[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.525221[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.322431[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.382325[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.368084[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.491047[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.184043[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.377993[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.492760[0m
[34m[1,0]<stdout>:## Epoch 33, Test Loss: 1.566704511642456, Test Accuracy: 43.75[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.348257[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.153920[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.398885[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.275753[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.329240[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.345312[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.254726[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.217169[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.243616[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.240882[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.319976[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.321837[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.267245[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.145490[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.333730[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.271563[0m
[34m[1,0]<stdout>:## Epoch 34, Test Loss: 1.580668568611145, Test Accuracy: 43.49300765991211[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.429609[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.584537[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.475435[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.499211[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.562497[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.488130[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.537476[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.475571[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.440558[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.519461[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.590365[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.472853[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.318921[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.500368[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.350610[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.501789[0m
[34m[1,0]<stdout>:## Epoch 35, Test Loss: 1.5397571325302124, Test Accuracy: 45.34333801269531[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.071959[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.317913[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.230347[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.251487[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.272609[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.216614[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.258913[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.295548[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.141388[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.335917[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.353179[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.169394[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.259986[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.180567[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.310315[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.339511[0m
[34m[1,0]<stdout>:## Epoch 36, Test Loss: 1.4876782894134521, Test Accuracy: 47.11143112182617[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.673500[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.665744[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.479038[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.441874[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.530367[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.497872[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.587842[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.496559[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.621074[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.405452[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.586010[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.402833[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.507040[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.660615[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.538106[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.672713[0m
[34m[1,0]<stdout>:## Epoch 37, Test Loss: 1.4821079969406128, Test Accuracy: 47.78988265991211[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.381592[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.474406[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.324642[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.327155[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.435691[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.444992[1,4]<stdout>:[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.404006[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.379212[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.375352[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.406904[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.462302[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.488958[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.492233[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.441364[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.469768[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.520664[0m
[34m[1,0]<stdout>:## Epoch 38, Test Loss: 1.5025135278701782, Test Accuracy: 46.361019134521484[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.625741[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.433024[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.727719[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.708473[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.525295[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.561512[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.553941[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.582765[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.422065[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.671246[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.546154[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.596296[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.663811[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.573056[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.622922[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.675926[0m
[34m[1,0]<stdout>:## Epoch 39, Test Loss: 1.5018876791000366, Test Accuracy: 46.26850128173828[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.553388[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.552845[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.620633[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.546280[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.479816[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.488529[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.449094[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.493062[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.455257[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.557738[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.457061[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.630175[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.426769[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.469432[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.304518[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.541117[0m
[34m[1,0]<stdout>:## Epoch 40, Test Loss: 1.4683438539505005, Test Accuracy: 46.74136734008789[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.337060[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.265386[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.271616[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.204854[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.320400[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.295924[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.212938[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.286291[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.272310[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.305922[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.279747[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.357652[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.297864[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.277241[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.246305[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.205405[0m
[34m[1,0]<stdout>:## Epoch 41, Test Loss: 1.4974067211151123, Test Accuracy: 46.607730865478516[1,0]<stdout>:[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.239461[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.363676[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.338900[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.277945[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.299646[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.397199[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.335924[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.249100[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.380498[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.309608[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.353211[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.363546[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.347355[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.429856[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.315624[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.297359[0m
[34m[1,0]<stdout>:## Epoch 42, Test Loss: 1.456189751625061, Test Accuracy: 48.550575256347656[1,0]<stdout>:[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.183627[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.209903[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.115427[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.166925[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.066567[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.045239[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.169032[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.130448[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.088316[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.122110[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.186498[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.024425[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.153332[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.214168[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.080055[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.124747[0m
[34m[1,0]<stdout>:## Epoch 43, Test Loss: 1.4529284238815308, Test Accuracy: 49.167354583740234[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.261793[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.125806[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.121035[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.178096[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.282347[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.159399[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.000526[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.311483[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.095858[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.070172[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.113625[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.232560[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.236951[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.181676[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.139397[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.159866[0m
[34m[1,0]<stdout>:## Epoch 44, Test Loss: 1.4242953062057495, Test Accuracy: 49.89720153808594[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.158644[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.182147[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.245021[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.215862[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.325443[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.176462[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.185174[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.307419[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.341313[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.123306[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.204859[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.277678[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.178976[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.319786[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.386050[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.279895[0m
[34m[1,0]<stdout>:## Epoch 45, Test Loss: 1.4022890329360962, Test Accuracy: 50.13363265991211[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.373753[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.264665[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.293780[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.361925[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.289642[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.287966[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.323026[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.395392[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.282153[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.294195[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.296920[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.377801[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.311090[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.204241[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.228473[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.233800[0m
[34m[1,0]<stdout>:## Epoch 46, Test Loss: 1.433719277381897, Test Accuracy: 50.04112243652344[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.385260[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.424966[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.326364[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.240111[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.338627[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.286153[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.276899[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.314758[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.203975[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.263325[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.274139[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.363636[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.268506[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.270187[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.288104[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.365111[0m
[34m[1,0]<stdout>:## Epoch 47, Test Loss: 1.4337129592895508, Test Accuracy: 49.732730865478516[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.222909[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.332367[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.283973[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.279783[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.351781[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.299432[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.353369[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.301148[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.571791[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.402988[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.391384[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.421052[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.337404[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.269217[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.436834[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.322491[0m
[34m[1,0]<stdout>:## Epoch 48, Test Loss: 1.4095920324325562, Test Accuracy: 50.42146301269531[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.136191[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.046071[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.348460[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.224502[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.098909[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.246811[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 1.206744[0m
[34m[1,6]<stdout>:## GPU6 - Step #0[1,6]<stdout>:#011Loss: 1.160488[1,6]<stdout>:[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.158477[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.095195[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 1.102270[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.254871[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.203737[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.100163[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.169326[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.149791[0m
[34m[1,0]<stdout>:## Epoch 49, Test Loss: 1.3828039169311523, Test Accuracy: 51.76809310913086[0m
[34m[1,0]<stdout>:## GPU0 - Step #0#011Loss: 1.021644[0m
[34m[1,3]<stdout>:## GPU3 - Step #0#011Loss: 1.092821[0m
[34m[1,2]<stdout>:## GPU2 - Step #0#011Loss: 1.096666[0m
[34m[1,1]<stdout>:## GPU1 - Step #0#011Loss: 1.046594[0m
[34m[1,5]<stdout>:## GPU5 - Step #0#011Loss: 1.086126[0m
[34m[1,4]<stdout>:## GPU4 - Step #0#011Loss: 1.032556[0m
[34m[1,7]<stdout>:## GPU7 - Step #0#011Loss: 0.973289[0m
[34m[1,6]<stdout>:## GPU6 - Step #0#011Loss: 1.068302[0m
[34m[1,9]<stdout>:## GPU9 - Step #0#011Loss: 1.078834[0m
[34m[1,13]<stdout>:## GPU13 - Step #0#011Loss: 1.109374[0m
[34m[1,15]<stdout>:## GPU15 - Step #0#011Loss: 1.287989[0m
[34m[1,14]<stdout>:## GPU14 - Step #0#011Loss: 1.020976[0m
[34m[1,12]<stdout>:## GPU12 - Step #0#011Loss: 1.059532[0m
[34m[1,8]<stdout>:## GPU8 - Step #0#011Loss: 1.038304[0m
[34m[1,10]<stdout>:## GPU10 - Step #0#011Loss: 0.999022[0m
[34m[1,11]<stdout>:## GPU11 - Step #0#011Loss: 1.044193[0m
[34m[1,13]<stdout>:Training Finished.[0m
[34m[1,13]<stdout>:[0m
[34m[1,13]<stdout>:################# Start Training ################[0m
[34m[1,10]<stdout>:Training Finished.[0m
[34m[1,10]<stdout>:[0m
[34m[1,10]<stdout>:################# Start Training ################[0m
[34m[1,1]<stdout>:Training Finished.[0m
[34m[1,1]<stdout>:[0m
[34m[1,1]<stdout>:################# Start Training ################[0m
[34m[1,8]<stdout>:Training Finished.[0m
[34m[1,8]<stdout>:[0m
[34m[1,8]<stdout>:################# Start Training ################[0m
[34m[1,11]<stdout>:Training Finished.[0m
[34m[1,11]<stdout>:[0m
[34m[1,11]<stdout>:################# Start Training ################[0m
[34m[1,14]<stdout>:Training Finished.[0m
[34m[1,14]<stdout>:[0m
[34m[1,14]<stdout>:################# Start Training ################[0m
[34m[1,15]<stdout>:Training Finished.[0m
[34m[1,15]<stdout>:[0m
[34m[1,15]<stdout>:################# Start Training ################[0m
[34m[1,9]<stdout>:Training Finished.[0m
[34m[1,9]<stdout>:[0m
[34m[1,9]<stdout>:################# Start Training ################[0m
[34m[1,12]<stdout>:Training Finished.[0m
[34m[1,12]<stdout>:[0m
[34m[1,12]<stdout>:################# Start Training ################[0m
[34m[1,5]<stdout>:Training Finished.[0m
[34m[1,5]<stdout>:[0m
[34m[1,5]<stdout>:################# Start Training ################[0m
[34m[1,2]<stdout>:Training Finished.[0m
[34m[1,2]<stdout>:[0m
[34m[1,2]<stdout>:################# Start Training ################[0m
[34m[1,4]<stdout>:Training Finished.[0m
[34m[1,4]<stdout>:[0m
[34m[1,4]<stdout>:################# Start Training ################[0m
[34m[1,6]<stdout>:Training Finished.[0m
[34m[1,6]<stdout>:[0m
[34m[1,6]<stdout>:################# Start Training ################[0m
[34m[1,7]<stdout>:Training Finished.[0m
[34m[1,7]<stdout>:[0m
[34m[1,7]<stdout>:################# Start Training ################[0m
[34m[1,3]<stdout>:Training Finished.[0m
[34m[1,3]<stdout>:[0m
[34m[1,3]<stdout>:################# Start Training ################[0m
[34m[1,0]<stdout>:## Epoch 50, Test Loss: 1.3374897241592407, Test Accuracy: 52.60074234008789[0m
[34m[1,0]<stdout>:Training Finished.[0m
[34m[1,0]<stdout>:[0m
[34m[1,0]<stdout>:################# Start Training ################[0m
[34m[1,0]<stdout>:[0m
[34m[1,0]<stdout>:################# Saving Model ################[0m
[34m[1,0]<stdout>:Model is saved in /opt/ml/model[0m
[35m2021-10-10 05:39:06,517 sagemaker-training-toolkit INFO Orted process exited[0m
[34mWarning: Permanently added 'algo-2,10.0.253.10' (ECDSA) to the list of known hosts.#015[0m
[34m[1,9]<stderr>:2021-10-10 05:34:14.589555: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m[1,9]<stderr>:2021-10-10 05:34:14.589709: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:105] SageMaker Profiler is not enabled. The timeline writer thread will not be started, future recorded events will be dropped.[0m
[34m[1,6]<stderr>:2021-10-10 05:34:14.620838: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m[1,6]<stderr>:2021-10-10 05:34:14.621026: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:105] SageMaker Profiler is not enabled. The timeline writer thread will not be started, future recorded events will be dropped.[0m
[34m[1,11]<stderr>:2021-10-10 05:34:14.639815: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m[1,11]<stderr>:2021-10-10 05:34:14.639990: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:105] SageMaker Profiler is not enabled. The timeline writer thread will not be started, future recorded events will be dropped.[0m
[34m[1,9]<stderr>:2021-10-10 05:34:14.641548: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m[1,6]<stderr>:2021-10-10 05:34:14.662533: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m[1,11]<stderr>:2021-10-10 05:34:14.682265: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m[1,4]<stderr>:2021-10-10 05:34:14.693571: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m[1,4]<stderr>:2021-10-10 05:34:14.693722: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:105] SageMaker Profiler is not enabled. The timeline writer thread will not be started, future recorded events will be dropped.[0m
[34m[1,5]<stderr>:2021-10-10 05:34:14.694566: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m[1,5]<stderr>:2021-10-10 05:34:14.694697: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:105] SageMaker Profiler is not enabled. The timeline writer thread will not be started, future recorded events will be dropped.[0m
[34m[1,12]<stderr>:2021-10-10 05:34:14.702525: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m[1,12]<stderr>:2021-10-10 05:34:14.702691: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:105] SageMaker Profiler is not enabled. The timeline writer thread will not be started, future recorded events will be dropped.[0m
[34m[1,15]<stderr>:2021-10-10 05:34:14.714861: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m[1,14]<stderr>:2021-10-10 05:34:14.714840: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m[1,14]<stderr>:2021-10-10 05:34:14.714970: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:105] SageMaker Profiler is not enabled. The timeline writer thread will not be started, future recorded events will be dropped.[0m
[34m[1,15]<stderr>:2021-10-10 05:34:14.714993: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:105] SageMaker Profiler is not enabled. The timeline writer thread will not be started, future recorded events will be dropped.[0m
[34m[1,3]<stderr>:2021-10-10 05:34:14.716759: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m[1,2]<stderr>:2021-10-10 05:34:14.716827: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m[1,3]<stderr>:2021-10-10 05:34:14.716970: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:105] SageMaker Profiler is not enabled. The timeline writer thread will not be started, future recorded events will be dropped.[0m
[34m[1,2]<stderr>:2021-10-10 05:34:14.716968: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:105] SageMaker Profiler is not enabled. The timeline writer thread will not be started, future recorded events will be dropped.[0m
[34m[1,1]<stderr>:2021-10-10 05:34:14.717599: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m[1,1]<stderr>:2021-10-10 05:34:14.717759: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:105] SageMaker Profiler is not enabled. The timeline writer thread will not be started, future recorded events will be dropped.[0m
[34m[1,7]<stderr>:2021-10-10 05:34:14.718411: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m[1,7]<stderr>:2021-10-10 05:34:14.718537: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:105] SageMaker Profiler is not enabled. The timeline writer thread will not be started, future recorded events will be dropped.[0m
[34m[1,10]<stderr>:2021-10-10 05:34:14.719183: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m[1,10]<stderr>:2021-10-10 05:34:14.719309: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:105] SageMaker Profiler is not enabled. The timeline writer thread will not be started, future recorded events will be dropped.[0m
[34m[1,3]<stderr>:2021-10-10 05:34:14.760081: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m[1,1]<stderr>:2021-10-10 05:34:14.760083: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m[1,7]<stderr>:2021-10-10 05:34:14.760082: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m[1,2]<stderr>:2021-10-10 05:34:14.760372: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m[1,13]<stderr>:2021-10-10 05:34:14.761591: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m[1,13]<stderr>:2021-10-10 05:34:14.762039: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:105] SageMaker Profiler is not enabled. The timeline writer thread will not be started, future recorded events will be dropped.[0m
[34m[1,4]<stderr>:2021-10-10 05:34:14.763771: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m[1,12]<stderr>:2021-10-10 05:34:14.763571: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m[1,5]<stderr>:2021-10-10 05:34:14.768281: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m[1,15]<stderr>:2021-10-10 05:34:14.779350: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m[1,14]<stderr>:2021-10-10 05:34:14.787810: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m[1,10]<stderr>:2021-10-10 05:34:14.798776: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m[1,13]<stderr>:2021-10-10 05:34:14.824711: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m[1,8]<stderr>:2021-10-10 05:34:15.289509: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m[1,8]<stderr>:2021-10-10 05:34:15.289690: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:105] SageMaker Profiler is not enabled. The timeline writer thread will not be started, future recorded events will be dropped.[0m
[34m[1,8]<stderr>:2021-10-10 05:34:15.330868: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m[1,0]<stderr>:2021-10-10 05:34:15.346388: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m[1,0]<stderr>:2021-10-10 05:34:15.346548: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:105] SageMaker Profiler is not enabled. The timeline writer thread will not be started, future recorded events will be dropped.[0m
[34m[1,0]<stderr>:2021-10-10 05:34:15.387990: W tensorflow/core/profiler/internal/smprofiler_timeline.cc:460] Initializing the SageMaker Profiler.[0m
[34m[1,0]<stderr>:2021-10-10 05:39:02.101411: W tensorflow/python/util/util.cc:348] Sets are not currently considered sequences, but this may change in the future, so consider avoiding using them.[0m
[34m[1,0]<stderr>:INFO:tensorflow:Assets written to: /opt/ml/model/1/assets[0m
[34m[1,0]<stderr>:INFO:tensorflow:Assets written to: /opt/ml/model/1/assets
[0m
[34m2021-10-10 05:39:06,503 sagemaker-training-toolkit INFO Reporting training SUCCESS[0m
2021-10-10 05:39:47 Uploading - Uploading generated training model
2021-10-10 05:39:47 Completed - Training job completed
[35m2021-10-10 05:39:36,547 sagemaker-training-toolkit INFO MPI process finished.[0m
[35m2021-10-10 05:39:36,548 sagemaker_tensorflow_container.training WARNING No model artifact is saved under path /opt/ml/model. Your training job will not save any model files to S3.[0m
[35mFor details of how to construct your training script see:[0m
[35mhttps://sagemaker.readthedocs.io/en/stable/using_tf.html#adapting-your-local-tensorflow-script[0m
[35m2021-10-10 05:39:36,548 sagemaker-training-toolkit INFO Reporting training SUCCESS[0m
Training seconds: 1042
Billable seconds: 1042
###Markdown
6. 정리 작업 모델 아티펙트 저장- S3 에 저장된 모델 아티펙트를 저장하여 추론시 사용합니다.
###Code
tf2_ddp_artifact_path = ddp_estimator.model_data
print("ddp_artifact_path: ", tf2_ddp_artifact_path)
%store tf2_ddp_artifact_path
! aws s3 ls {tf2_ddp_artifact_path} --recursive
###Output
2021-10-10 05:39:42 6052287 cifar10-sm-ddp-2021-10-10-05-27-44-881/output/model.tar.gz
|
worksheets/.ipynb_checkpoints/Week 03 - Worksheet 1 - Syntax - Data structures (tuples lists and slices)-checkpoint.ipynb | ###Markdown
Week 02, Worksheet 1: Data structures (tuples, lists, and "slices") To use this worksheet, click the Kernel menu and click Restart Kernel and Run All Cells to run the code below to set up the activity at the end. Otherwise, you can press SHIFT + ENTER in each code cell to run the code to prepare it. `tuple` or not `tuple`: that is the questionLet's jump right in and have a look at a `tuple`:
###Code
integers = (0, 1, 2, 3, 4, 5, 6, 7, 8, 9)
print(integers)
###Output
_____no_output_____
###Markdown
You're probably reading this worksheet and thinking to yourself "OK, `tuple`s are basically lists. So what gives?" You're right, `tuple`s are like lists. They behave the same way _except_ that `tuple`s can't be modified in the same way. In addition, there are a few small differences in how we might use them:1. there is no `del` function that we can use on a tuple2. we can't `append` anything to them, though through some trickery we can `+` to themSo what are `tuple`s used for? Well, quite a few things that we'll see in the future, and quite a few things that you've already done. For example, any time we pass `arguments` to a `function` or a `method`, we're really using a secret `tuple`.Conveniently, we can test some of our `list` skills with them, and expand our knowledge of _data structures_. So, let's review a bit from our last worksheet. `print` each of the following: 1. The 6th item in the `integers` `tuple`.Hint: this is tricky because I'm asking for the 6th item.
###Code
print(integers[5])
###Output
_____no_output_____
###Markdown
2. What about the 1st item in the `tuple`?
###Code
print(integers[0])
###Output
0
###Markdown
3. What's the sum of the 2nd, 3rd, and 9th item in the `tuple`?
###Code
print(integers[1] + integers[2] + integers[8])
###Output
11
###Markdown
Joining `tuple`sAs referenced above, there is a sneaky way we can _add_ to `tuple`s. Just like the way the `+` operator works with number and `string` types, we can use the operator to _join_ tuples:
###Code
negative_integers = (-9, -8, -7, -6, -5, -4, -3, -2, -1)
integers = negative_integers + integers
print(integers)
###Output
(-9, -8, -7, -6, -5, -4, -3, -2, -1, 0, 1, 2, 3, 4, 5, 6, 7, 8, 9)
###Markdown
What else so `tuple`s and `list`s have in common?One feature that we didn't talk about in the `list`s worksheet is something referred to as "slicing." This gives us the ability to get parts of a `tuple` or `list`. We're already familiar with `index`es:
###Code
# An example of an index
cat_names = ["Ulysses", "Snooze Magoo", "Mr. U", "The Boss"]
print("My cat's name: ", cat_names[0]) # That's his real name, but we call him that the least
###Output
My cat's name: Ulysses
###Markdown
Honestly, I think my cat has forgotten his name. We refer to him by the other three more often. So, let's extract those out into a variable I'm going to call `common_cat_names` using _slicing syntax_:
###Code
# Here, [1:] means "index 1 to the end
common_cat_names = cat_names[1:]
print(common_cat_names)
###Output
['Snooze Magoo', 'Mr. U', 'The Boss']
###Markdown
But, we can slice this any number of ways, including _backwards_:
###Code
# The first three
print("First three:", cat_names[:3])
# A slice starting at position one and stopping, not including, position 3
print("Start at one, stop before position 3:", cat_names[1:3])
# Every two
print("Evens:", cat_names[1::2])
print("Odds:", cat_names[0::2])
# Backwards
print("Backwards:", cat_names[::-1])
###Output
First three: ['Ulysses', 'Snooze Magoo', 'Mr. U']
Start at one, stop before position 3: ['Snooze Magoo', 'Mr. U']
Evens: ['Snooze Magoo', 'The Boss']
Odds: ['Ulysses', 'Mr. U']
Backwards: ['The Boss', 'Mr. U', 'Snooze Magoo', 'Ulysses']
###Markdown
Negative indexingThe last one points at something else we can do: _negative indexing_, that is start counting from the _end_ of the `list` or `tuple`.
###Code
# Get the last value
print(cat_names[-1])
# Get the second to last value
print(cat_names[-2])
###Output
The Boss
Mr. U
###Markdown
Test driveLet's perform some operations on our variable `integers`. `print` the result of each of the following operations: 1. A slice starting at position 3 and continuing until position 10.
###Code
print(integers[3:10])
print(integers[1::2])
print(integers[0::2])
print(integers[::-1])
print(integers[::-3])
###Output
(-6, -5, -4, -3, -2, -1, 0)
(-8, -6, -4, -2, 0, 2, 4, 6, 8)
(-9, -7, -5, -3, -1, 1, 3, 5, 7, 9)
(9, 8, 7, 6, 5, 4, 3, 2, 1, 0, -1, -2, -3, -4, -5, -6, -7, -8, -9)
(9, 6, 3, 0, -3, -6, -9)
|
book/pandas/01-Introduction to Pandas.ipynb | ###Markdown
Table of Contents1 What is Pandas?2 Pandas Series3 Pandas DataFrame4 Advantages of Pandas5 Creating Series6 Creating DataFrame6.1 About the Author What is Pandas?The Pandas library is built on NumPy and provides easy-to-use data structures and data analysis tools for the Python programming language. Pandas Series A **one-dimensional** labeled array a capable of holding any data type Pandas DataFrame A **two-dimensional** labeled data structure with columns of potentially different types Advantages of Pandas - Data representation- Less writing and more work done- An extensive set of features- Efficiently handles large data- Makes data flexible and customizable- Made for Python
###Code
# Conventional way to import pandas
import pandas as pd
# Check pandas version
pd.__version__
# Show version of all packages
pd.show_versions()
###Output
INSTALLED VERSIONS
------------------
commit : None
python : 3.7.4.final.0
python-bits : 64
OS : Linux
OS-release : 5.3.0-26-generic
machine : x86_64
processor : x86_64
byteorder : little
LC_ALL : None
LANG : en_US.UTF-8
LOCALE : en_US.UTF-8
pandas : 0.25.1
numpy : 1.17.2
pytz : 2019.3
dateutil : 2.8.0
pip : 19.2.3
setuptools : 41.4.0
Cython : 0.29.13
pytest : 5.2.1
hypothesis : None
sphinx : 2.2.0
blosc : None
feather : None
xlsxwriter : 1.2.1
lxml.etree : 4.4.1
html5lib : 1.0.1
pymysql : None
psycopg2 : None
jinja2 : 2.10.3
IPython : 7.8.0
pandas_datareader: None
bs4 : 4.8.0
bottleneck : 1.2.1
fastparquet : None
gcsfs : None
lxml.etree : 4.4.1
matplotlib : 3.1.1
numexpr : 2.7.0
odfpy : None
openpyxl : 3.0.0
pandas_gbq : None
pyarrow : None
pytables : None
s3fs : None
scipy : 1.3.1
sqlalchemy : 1.3.9
tables : 3.5.2
xarray : None
xlrd : 1.2.0
xlwt : 1.3.0
xlsxwriter : 1.2.1
###Markdown
Creating Series
###Code
# Create Series
s1 = pd.Series([3, 6, 9, 12])
s1
# Check type
type(s1)
# To see values
s1.values
# To see index/keys
s1.index
# Creating labeled series
s2 = pd.Series([200000, 300000, 4000000, 500000], index=['A', 'B', 'C', 'D'])
s2
s2.values
s2.index
# Indexing
s2['A']
# Boolean indexing
s2[s2 > 700000]
###Output
_____no_output_____
###Markdown
Creating DataFrame
###Code
# Create a DataFrame
data = {'Country': ['Belgium', 'India', 'Brazil'],
'Capital': ['Brussels', 'New Delhi', 'Brasília'],
'Population': [11190846, 1303171035, 207847528]
}
df = pd.DataFrame(data, columns=["Country", "Capital", "Population"])
df
# Check type
type(df)
# Indexing
df["Country"]
# or
df.Country
# Boolean indexing
df["Population"] > 40000000
df["Country"] == "Belgium"
df["Capital"] == "Brasilia"
###Output
_____no_output_____ |
UNICEF Arm 2030 VISION #1 Flood Prediction in Malawi/Solution 1/lili-checkpoint.ipynb | ###Markdown
Importing the Necessary Libraries
###Code
import pandas as pd
import lightgbm as lgb
import numpy as np
###Output
_____no_output_____
###Markdown
numpy version '1.18.2'
###Code
np.__version__
###Output
_____no_output_____
###Markdown
pandas version '1.0.1'
###Code
pd.__version__
###Output
_____no_output_____
###Markdown
lightgbm version 2.3.1
###Code
!pip install lightgbm==2.3.1
lgb.__version__
###Output
Requirement already satisfied: lightgbm==2.3.1 in d:\conda\lib\site-packages (2.3.1)
Requirement already satisfied: numpy in d:\conda\lib\site-packages (from lightgbm==2.3.1) (1.18.2)
Requirement already satisfied: scipy in d:\conda\lib\site-packages (from lightgbm==2.3.1) (1.4.1)
Requirement already satisfied: scikit-learn in d:\conda\lib\site-packages (from lightgbm==2.3.1) (0.22.1)
Requirement already satisfied: joblib>=0.11 in d:\conda\lib\site-packages (from scikit-learn->lightgbm==2.3.1) (0.14.1)
###Markdown
Reading the Data
###Code
sub = pd.read_csv('SampleSubmission (1).csv')
df=pd.read_csv('Train.csv')
###Output
_____no_output_____
###Markdown
Data Cleaning for this part I have used the start code: https://github.com/DariusTheGeek/Flood-Prediction-in-Malawi--Zindi-Competition to prepare the train and the test
###Code
precip_features_2019 = []
precip_features_2015 = []
for col in df.columns:
if '2019' in col:
precip_features_2019.append(col)
elif 'precip 2014' in col:
precip_features_2015.append(col)
elif 'precip 2015' in col:
precip_features_2015.append(col)
train=tain = df[df.columns.difference(precip_features_2019)]
precip_features_2019.extend(['X', 'Y', 'elevation', 'LC_Type1_mode', 'Square_ID'])
test = df[precip_features_2019]
new_2015_cols = {}
for col, number in zip(precip_features_2015, range(1, len(precip_features_2015) + 1)):
if 'precip' in col:
new_2015_cols[col] = 'week_' + str(number) + '_precip'
new_2019_cols = {}
for col, number in zip(precip_features_2019, range(1, len(precip_features_2019) + 1)):
if 'precip' in col:
new_2019_cols[col] = 'week_' + str(number) + '_precip'
train.rename(columns = new_2015_cols, inplace = True)
test.rename(columns = new_2019_cols, inplace = True)
train=pd.concat([tain[:12000],train[12000:][train[12000:]['target_2015']<0.1]])
target = train.target_2015
train, test = train.align(test, join = 'inner', axis = 1)
from sklearn.model_selection import train_test_split
from sklearn.metrics import mean_squared_error,mean_absolute_error
X = train.drop(['Square_ID'], axis = 1)
y = target
###Output
D:\conda\lib\site-packages\pandas\core\frame.py:4133: SettingWithCopyWarning:
A value is trying to be set on a copy of a slice from a DataFrame
See the caveats in the documentation: https://pandas.pydata.org/pandas-docs/stable/user_guide/indexing.html#returning-a-view-versus-a-copy
errors=errors,
###Markdown
key point: The flood may occur in consequence of heavy precipitation that occur during a period less than 18 weeks. For that reason we have just worked on the period with a continuios heavy precip.
###Code
train[train.columns[5:-1]].describe().loc[['mean']]
###Output
_____no_output_____
###Markdown
Max precip within 2 weeks
###Code
train['week_7_precip_']=train['week_7_precip']+train['week_6_precip']
test['week_7_precip_']=test['week_7_precip']+test['week_6_precip']
train['week_8_precip_']=train['week_8_precip']+train['week_7_precip']
test['week_8_precip_']=test['week_8_precip']+test['week_7_precip']
train['week_9_precip_']=train['week_9_precip']+train['week_8_precip']
test['week_9_precip_']=test['week_9_precip']+test['week_8_precip']
train['max_2_weeks']=train[['week_7_precip_','week_8_precip_','week_9_precip_']].apply(np.max,axis=1)
test['max_2_weeks']=test[['week_7_precip_','week_8_precip_','week_9_precip_']].apply(np.max,axis=1)
X1=train[['LC_Type1_mode', 'X', 'Y', 'elevation','week_7_precip', 'week_8_precip', 'week_9_precip','max_2_weeks']]
sub1=test[['LC_Type1_mode', 'X', 'Y', 'elevation','week_7_precip', 'week_8_precip', 'week_9_precip','max_2_weeks']]
X1.columns=sub1.columns
###Output
_____no_output_____
###Markdown
Week within the max of precip
###Code
def index(col):
l=list(col)
return l.index(max(l))
X1['max_index']=train[['week_6_precip', 'week_7_precip', 'week_8_precip','week_9_precip']].apply(index,axis=1)
sub1['max_index']=test[['week_6_precip', 'week_7_precip', 'week_8_precip','week_9_precip']].apply(index,axis=1)
###Output
D:\conda\lib\site-packages\ipykernel_launcher.py:5: SettingWithCopyWarning:
A value is trying to be set on a copy of a slice from a DataFrame.
Try using .loc[row_indexer,col_indexer] = value instead
See the caveats in the documentation: https://pandas.pydata.org/pandas-docs/stable/user_guide/indexing.html#returning-a-view-versus-a-copy
"""
D:\conda\lib\site-packages\ipykernel_launcher.py:6: SettingWithCopyWarning:
A value is trying to be set on a copy of a slice from a DataFrame.
Try using .loc[row_indexer,col_indexer] = value instead
See the caveats in the documentation: https://pandas.pydata.org/pandas-docs/stable/user_guide/indexing.html#returning-a-view-versus-a-copy
###Markdown
Precip variation over different weeks
###Code
sub1['slope_8_7']=((test['week_8_precip']/test['week_7_precip'])>1)*1
X1['slope_8_7']=((train['week_8_precip']/train['week_7_precip'])>1)*1
sub1['slope_9_8']=((test['week_9_precip']/test['week_8_precip'])>1)*1
X1['slope_9_8']=((train['week_9_precip']/train['week_8_precip'])>1)*1
###Output
D:\conda\lib\site-packages\ipykernel_launcher.py:1: SettingWithCopyWarning:
A value is trying to be set on a copy of a slice from a DataFrame.
Try using .loc[row_indexer,col_indexer] = value instead
See the caveats in the documentation: https://pandas.pydata.org/pandas-docs/stable/user_guide/indexing.html#returning-a-view-versus-a-copy
"""Entry point for launching an IPython kernel.
D:\conda\lib\site-packages\ipykernel_launcher.py:2: SettingWithCopyWarning:
A value is trying to be set on a copy of a slice from a DataFrame.
Try using .loc[row_indexer,col_indexer] = value instead
See the caveats in the documentation: https://pandas.pydata.org/pandas-docs/stable/user_guide/indexing.html#returning-a-view-versus-a-copy
D:\conda\lib\site-packages\ipykernel_launcher.py:5: SettingWithCopyWarning:
A value is trying to be set on a copy of a slice from a DataFrame.
Try using .loc[row_indexer,col_indexer] = value instead
See the caveats in the documentation: https://pandas.pydata.org/pandas-docs/stable/user_guide/indexing.html#returning-a-view-versus-a-copy
"""
D:\conda\lib\site-packages\ipykernel_launcher.py:6: SettingWithCopyWarning:
A value is trying to be set on a copy of a slice from a DataFrame.
Try using .loc[row_indexer,col_indexer] = value instead
See the caveats in the documentation: https://pandas.pydata.org/pandas-docs/stable/user_guide/indexing.html#returning-a-view-versus-a-copy
###Markdown
outlier detection
###Code
from sklearn.covariance import EllipticEnvelope
clf2 = EllipticEnvelope(contamination=.17,random_state=0)
clf2.fit(X1)
ee_scores = pd.Series(clf2.decision_function(X1))
clusters2 = clf2.predict(X1)
X1['target']=target
X1['pred']=clusters2
X1=X1[X1['pred']!=-1]
X1,y=X1.drop(columns=['pred','target']),X1['target']
###Output
D:\conda\lib\site-packages\sklearn\covariance\_robust_covariance.py:170: RuntimeWarning: Determinant has increased; this should not happen: log(det) > log(previous_det) (-62.156597799790795 > -63.517750296664858). You may want to try with a higher value of support_fraction (current value: 0.503).
RuntimeWarning)
D:\conda\lib\site-packages\sklearn\covariance\_robust_covariance.py:170: RuntimeWarning: Determinant has increased; this should not happen: log(det) > log(previous_det) (-60.551761565457447 > -61.007147944574804). You may want to try with a higher value of support_fraction (current value: 0.503).
RuntimeWarning)
D:\conda\lib\site-packages\sklearn\covariance\_robust_covariance.py:170: RuntimeWarning: Determinant has increased; this should not happen: log(det) > log(previous_det) (-61.346057144722828 > -61.944318737644792). You may want to try with a higher value of support_fraction (current value: 0.501).
RuntimeWarning)
D:\conda\lib\site-packages\ipykernel_launcher.py:6: SettingWithCopyWarning:
A value is trying to be set on a copy of a slice from a DataFrame.
Try using .loc[row_indexer,col_indexer] = value instead
See the caveats in the documentation: https://pandas.pydata.org/pandas-docs/stable/user_guide/indexing.html#returning-a-view-versus-a-copy
D:\conda\lib\site-packages\ipykernel_launcher.py:7: SettingWithCopyWarning:
A value is trying to be set on a copy of a slice from a DataFrame.
Try using .loc[row_indexer,col_indexer] = value instead
See the caveats in the documentation: https://pandas.pydata.org/pandas-docs/stable/user_guide/indexing.html#returning-a-view-versus-a-copy
import sys
###Markdown
using Soil organic carbon stock: (external data): extrcted from "https://soilgrids.org/" mapped using QGIS (majority)
###Code
s=pd.read_csv('ss.csv')
s.drop(columns='_soilvarie',axis=1,inplace=True)
X1=X1.merge(s,on=['X','Y'],how='left')
sub1=sub1.merge(s,on=['X','Y'],how='left')
def metric(predictions, targets):
return np.sqrt(((predictions - targets) ** 2).mean())
###Output
_____no_output_____
###Markdown
Training model
###Code
params = { 'learning_rate':0.07,'max_depth':8}
X=X1
X_test=sub1
n_estimators = 221
n_iters = 5
preds_buf = []
err_buf = []
for i in range(n_iters):
x_train, x_valid, y_train, y_valid = train_test_split(X, y, test_size=0.2, random_state=i)
d_train = lgb.Dataset(x_train, label=y_train)
d_valid = lgb.Dataset(x_valid, label=y_valid)
watchlist = [d_valid]
model = lgb.train(params, d_train, n_estimators, watchlist, verbose_eval=1)
preds = model.predict(x_valid)
err_buf.append(metric(model.predict(x_valid),y_valid))
preds = model.predict(X_test)
preds_buf.append(preds)
print('Mean RMSLE = ' + str(np.mean(err_buf)) + ' +/- ' + str(np.std(err_buf)))
# Average predictions
preds1 = np.mean(preds_buf, axis=0)
def check(col):
if col<0:
return 0
elif col>1:
return 1
else:
return col
preds=preds1
preds-=0.08
submission_df = pd.DataFrame({'Square_ID': test.Square_ID, 'target_2019': preds})
submission_df['target_2019']=submission_df['target_2019'].apply(check)
submission_df.to_csv('lili.csv', index = False)
###Output
_____no_output_____ |
section5/Lecture40_DeepNN_Creditcard_dataset.ipynb | ###Markdown
Importing and visualizing dataset
###Code
#import the dataset
iris = pd.read_csv("creditcard.csv")
iris.head()
###Output
_____no_output_____
###Markdown
Seperating Examples and Labels
###Code
X= iris.iloc[:, 1:29]
Y= iris.iloc[:, 30]
from sklearn.preprocessing import normalize
X = normalize(X)
y = np.array(Y)
y = y.astype(int)
X_train,X_test,Y_train,Y_test= train_test_split(X, y, test_size= 0.10, random_state= 1)
print ('X_train shape: ',X_train.shape)
print ('y_train shape: ',Y_train.shape)
print ('X_test shape: ',X_test.shape)
print ('y_test shape: ',Y_test.shape)
###Output
X_train shape: (256326, 28)
y_train shape: (256326,)
X_test shape: (28481, 28)
y_test shape: (28481,)
###Markdown
Using Dataloader to convert numpy arrays to Tensors
###Code
trainloader = DataLoader(TensorDataset(torch.from_numpy(X_train), torch.from_numpy(Y_train)),
batch_size=len(X_train), shuffle=True)
testloader = DataLoader(TensorDataset(torch.from_numpy(X_test), torch.from_numpy(Y_test)),
batch_size=len(X_test), shuffle=False)
dataloaders = {
"train": trainloader,
"validation": testloader
}
###Output
_____no_output_____
###Markdown
This class will define our model Using __init__ we will define numbers of nodes in our particular layer Using forward() we will define functionality of each layer
###Code
class Classifier(nn.Module):
def __init__(self):
super().__init__()
self.fc1 = nn.Linear(28, 340)
self.fc2 = nn.Linear(340, 220)
self.fc3 = nn.Linear(220, 200)
self.fc4 = nn.Linear(200, 70)
self.fc5 = nn.Linear(70, 10)
self.fc6 = nn.Linear(10, 2)
self.dropout = nn.Dropout(p=0.2)
def forward(self, x):
#x = x.view(x.shape[0], -1)
#print(x)
x = F.relu(self.fc1(x))
x = F.relu(self.fc2(x))
x = F.relu(self.fc3(x))
x = F.relu(self.fc4(x))
x = self.dropout(F.relu(self.fc5(x)))
x = F.log_softmax(self.fc6(x), dim=1)
#x = F.log_softmax(self.fc4(x))
return x
###Output
_____no_output_____
###Markdown
Model declaration, Type of loss and optimizer. We are using adam optimizer to optimize our network
###Code
model = Classifier()
criterion = nn.NLLLoss()
optimizer = optim.Adam(model.parameters(), lr=0.01)
###Output
_____no_output_____
###Markdown
This block is showing summary off our model
###Code
model
###Output
_____no_output_____
###Markdown
This function is predicting output of examples we will feed in. Will be useful in calculating model accuracies.
###Code
def predict(model, inputs):
output = model(inputs)
return output.data.numpy().argmax(axis= 1)
###Output
_____no_output_____
###Markdown
Here we will perform forward and backward propagation.
###Code
from torch.autograd import Variable
loss1=[]
train_acc=[]
Epoch=40
for epoch in range(Epoch):
print('------------------------------------------------------------------------------------------')
acc=0
train_acc1=0
for i, (features, labels) in enumerate(trainloader):
#print(features.shape)
features = Variable(features)
labels = Variable(labels)
optimizer.zero_grad()
features=features.float()
outputs = model(features)
loss = criterion(outputs, labels.long())
loss.backward()
optimizer.step()
if (i+1) % len(trainloader) == 0:
Ypred = predict(model, torch.from_numpy(X_train).float())
acc = np.mean(Y_train == Ypred)
# train_acc1=train_accuracy/len(trainloader)
train_acc1=acc/len(trainloader)
train_acc.append(train_acc1)
loss1.append(loss.data)
print ('Epoch [%d/%d], Iter [%d] Loss: %.4f Training Accuracy: %.5f' %(epoch+1, 40, i+1, loss.data, train_acc1 ))
###Output
------------------------------------------------------------------------------------------
Epoch [1/40], Iter [1] Loss: 0.7079 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [2/40], Iter [1] Loss: 0.6593 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [3/40], Iter [1] Loss: 0.5594 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [4/40], Iter [1] Loss: 0.3087 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [5/40], Iter [1] Loss: 0.0671 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [6/40], Iter [1] Loss: 0.0211 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [7/40], Iter [1] Loss: 0.0274 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [8/40], Iter [1] Loss: 0.0398 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [9/40], Iter [1] Loss: 0.0505 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [10/40], Iter [1] Loss: 0.0555 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [11/40], Iter [1] Loss: 0.0558 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [12/40], Iter [1] Loss: 0.0501 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [13/40], Iter [1] Loss: 0.0461 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [14/40], Iter [1] Loss: 0.0391 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [15/40], Iter [1] Loss: 0.0351 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [16/40], Iter [1] Loss: 0.0299 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [17/40], Iter [1] Loss: 0.0280 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [18/40], Iter [1] Loss: 0.0246 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [19/40], Iter [1] Loss: 0.0208 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [20/40], Iter [1] Loss: 0.0179 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [21/40], Iter [1] Loss: 0.0181 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [22/40], Iter [1] Loss: 0.0150 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [23/40], Iter [1] Loss: 0.0144 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [24/40], Iter [1] Loss: 0.0126 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [25/40], Iter [1] Loss: 0.0111 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [26/40], Iter [1] Loss: 0.0103 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [27/40], Iter [1] Loss: 0.0094 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [28/40], Iter [1] Loss: 0.0088 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [29/40], Iter [1] Loss: 0.0088 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [30/40], Iter [1] Loss: 0.0084 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [31/40], Iter [1] Loss: 0.0082 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [32/40], Iter [1] Loss: 0.0081 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [33/40], Iter [1] Loss: 0.0078 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [34/40], Iter [1] Loss: 0.0077 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [35/40], Iter [1] Loss: 0.0077 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [36/40], Iter [1] Loss: 0.0072 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [37/40], Iter [1] Loss: 0.0068 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [38/40], Iter [1] Loss: 0.0065 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [39/40], Iter [1] Loss: 0.0062 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [40/40], Iter [1] Loss: 0.0061 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [41/40], Iter [1] Loss: 0.0059 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [42/40], Iter [1] Loss: 0.0059 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [43/40], Iter [1] Loss: 0.0056 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [44/40], Iter [1] Loss: 0.0059 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [45/40], Iter [1] Loss: 0.0055 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [46/40], Iter [1] Loss: 0.0055 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [47/40], Iter [1] Loss: 0.0054 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [48/40], Iter [1] Loss: 0.0053 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [49/40], Iter [1] Loss: 0.0051 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [50/40], Iter [1] Loss: 0.0048 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [51/40], Iter [1] Loss: 0.0047 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [52/40], Iter [1] Loss: 0.0047 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [53/40], Iter [1] Loss: 0.0046 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [54/40], Iter [1] Loss: 0.0043 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [55/40], Iter [1] Loss: 0.0043 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [56/40], Iter [1] Loss: 0.0044 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [57/40], Iter [1] Loss: 0.0044 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
Epoch [58/40], Iter [1] Loss: 0.0045 Training Accuracy: 0.99827
------------------------------------------------------------------------------------------
###Markdown
we will plot our accuracies and loss functions below.
###Code
np_loss=loss1[0].numpy()
for i in range(len(loss1)):
np_loss=np.append(np_loss, loss1[i])
np_acc=0.02
for i in range(len(train_acc)):
np_acc=np.append(np_acc, train_acc[i])
###Output
_____no_output_____
###Markdown
Training Accuracy
###Code
%matplotlib inline
plt.plot(np_acc, color='blue')
plt.title("Training Accuracy")
plt.show()
###Output
_____no_output_____
###Markdown
Training Loss
###Code
%matplotlib inline
plt.plot(np_loss, color='red', label='Trainig loss')
plt.title("Traininng Loss")
plt.legend()
plt.show()
###Output
_____no_output_____
###Markdown
Training loss and accuracy curves
###Code
%matplotlib inline
plt.plot(np_loss, color='red', label='Trainig loss')
plt.plot(np_acc, color='blue', label='Training Accuracy')
plt.title("Loss/Accuracy curves")
plt.legend()
plt.show()
###Output
_____no_output_____
###Markdown
Model test accuracy
###Code
Ypred = predict(model, torch.from_numpy(X_test).float())
acc = np.mean(Y_test == Ypred)
print('Test accuracy: ', acc)
###Output
Test accuracy: 0.9982795547909132
###Markdown
Precision/ Recall / F1 Scores using sklearn
###Code
from sklearn.metrics import classification_report
target_names = ['Class 0', 'Class 1']
print(classification_report(Y_test, Ypred, target_names=target_names))
###Output
C:\Users\wajhi\Anaconda3\lib\site-packages\sklearn\metrics\classification.py:1437: UndefinedMetricWarning: Precision and F-score are ill-defined and being set to 0.0 in labels with no predicted samples.
'precision', 'predicted', average, warn_for)
|
finch/python/kbqa/regex.ipynb | ###Markdown
Knowledge
###Code
URI_PREFIX = 'http://kgdemo.com/'
triples = [
('宝马', 'is_what', '宝马(BMW)是德国豪华汽车品牌'),
('宝马', 'is_how', '德系大品牌值得信赖,各方面口碑都很好'),
('宝马', 'is_compared', '各有千秋,但是人生苦短,我选宝马'),
('捷豹', 'is_what', '捷豹(Jaguar)英国豪华汽车品牌,英国皇室御用品牌,1935年诞生'),
('宾利', 'is_how', '举世闻名的豪华汽车制造品牌,非常昂贵哦'),
('帕加尼', 'is_what', '帕加尼(Pagani)是一家位于意大利摩德纳的超级跑车制造商,该车厂坚持手工打造车辆,其汽车产量非常少,价格也十分昂贵'),
('广汽本田', 'is_what', '广汽本田汽车有限公司(原广州本田汽车有限公司;简称广汽本田)于1998年7月1日成立,它是由广州汽车集团公司与日本本田技研工业株式会社共同出资组建的合资公司,双方各占50%股份,合作年限为30年'),
('北京奔驰', 'is_how', '大品牌值得信赖,我经常在宝马的后视镜里看到它'),
]
graph = rdflib.Graph()
resources = set([r for triple in triples for r in triple])
resource2uri = {r: URI_PREFIX + r for r in resources}
uri2resource = {uri: r for r, uri in resource2uri.items()}
for (s, p, o) in triples:
s_uri = rdflib.URIRef(resource2uri[s])
p_uri = rdflib.URIRef(resource2uri[p])
o_uri = rdflib.URIRef(resource2uri[o])
graph.add((s_uri, p_uri, o_uri))
###Output
_____no_output_____
###Markdown
Rule
###Code
class Intent(object):
CarIsWhat = 'car_is_what'
CarIsHow = 'car_is_how'
CarIsCompared = 'car_is_compared'
rules = [
(Intent.CarIsWhat, r"(?:我想|给我)(?:了解|介绍|讲解)(?:一下)?(.*)(?:这个汽车品牌)"),
(Intent.CarIsWhat, r"(?:我想|给我)(?:了解|介绍|讲解)(?:一下)?(.*)$"),
(Intent.CarIsCompared, r"(.*)和.*比(?:怎么样|哪个好|哪个更好)"),
(Intent.CarIsHow, r"(.*?)(?:这个牌子的汽车|这个汽车品牌|汽车)?(?:怎么样|好用吗|如何呢)"),
(Intent.CarIsWhat, r"(.*)是什么"),
]
def retrieve_kg(graph, db_query, uri2resource):
print('SPARQL:')
print(db_query)
for row in graph.query(db_query):
print('Output:', uri2resource[row.o.toPython()])
print()
print()
for utt in ['宝马是什么',
'我想了解一下宝马',
'给我介绍一下宝马',
'给我讲解一下捷豹这个汽车品牌',
'给我介绍一下帕加尼',
'我想了解一下广汽本田',
'宝马这个牌子的汽车怎么样',
'宾利这个牌子的汽车怎么样',
'北京奔驰怎么样',
'宝马如何呢',
'宝马汽车好用吗',
'宝马和奔驰比怎么样',
'宝马和奔驰比哪个好',
'宝马和奔驰比哪个更好',]:
print(utt)
for intent, rule in rules:
slots = re.findall(rule, utt)
if len(slots) > 0:
print('Intent:', intent)
print('Rule:', rule)
for slot in slots:
if intent == Intent.CarIsWhat:
db_query = (
"""
PREFIX : <%s>
SELECT DISTINCT ?o WHERE {
:%s :is_what ?o .
}
""" % (URI_PREFIX, slot)
)
retrieve_kg(graph, db_query, uri2resource)
if intent == Intent.CarIsHow:
db_query = (
"""
PREFIX : <%s>
SELECT DISTINCT ?o WHERE {
:%s :is_how ?o .
}
""" % (URI_PREFIX, slot)
)
retrieve_kg(graph, db_query, uri2resource)
if intent == Intent.CarIsCompared:
db_query = (
"""
PREFIX : <%s>
SELECT DISTINCT ?o WHERE {
:%s :is_compared ?o .
}
""" % (URI_PREFIX, slot)
)
retrieve_kg(graph, db_query, uri2resource)
break
###Output
宝马是什么
Intent: car_is_what
Rule: (.*)是什么
SPARQL:
PREFIX : <http://kgdemo.com/>
SELECT DISTINCT ?o WHERE {
:宝马 :is_what ?o .
}
Output: 宝马(BMW)是德国豪华汽车品牌
我想了解一下宝马
Intent: car_is_what
Rule: (?:我想|给我)(?:了解|介绍|讲解)(?:一下)?(.*)$
SPARQL:
PREFIX : <http://kgdemo.com/>
SELECT DISTINCT ?o WHERE {
:宝马 :is_what ?o .
}
Output: 宝马(BMW)是德国豪华汽车品牌
给我介绍一下宝马
Intent: car_is_what
Rule: (?:我想|给我)(?:了解|介绍|讲解)(?:一下)?(.*)$
SPARQL:
PREFIX : <http://kgdemo.com/>
SELECT DISTINCT ?o WHERE {
:宝马 :is_what ?o .
}
Output: 宝马(BMW)是德国豪华汽车品牌
给我讲解一下捷豹这个汽车品牌
Intent: car_is_what
Rule: (?:我想|给我)(?:了解|介绍|讲解)(?:一下)?(.*)(?:这个汽车品牌)
SPARQL:
PREFIX : <http://kgdemo.com/>
SELECT DISTINCT ?o WHERE {
:捷豹 :is_what ?o .
}
Output: 捷豹(Jaguar)英国豪华汽车品牌,英国皇室御用品牌,1935年诞生
给我介绍一下帕加尼
Intent: car_is_what
Rule: (?:我想|给我)(?:了解|介绍|讲解)(?:一下)?(.*)$
SPARQL:
PREFIX : <http://kgdemo.com/>
SELECT DISTINCT ?o WHERE {
:帕加尼 :is_what ?o .
}
Output: 帕加尼(Pagani)是一家位于意大利摩德纳的超级跑车制造商,该车厂坚持手工打造车辆,其汽车产量非常少,价格也十分昂贵
我想了解一下广汽本田
Intent: car_is_what
Rule: (?:我想|给我)(?:了解|介绍|讲解)(?:一下)?(.*)$
SPARQL:
PREFIX : <http://kgdemo.com/>
SELECT DISTINCT ?o WHERE {
:广汽本田 :is_what ?o .
}
Output: 广汽本田汽车有限公司(原广州本田汽车有限公司;简称广汽本田)于1998年7月1日成立,它是由广州汽车集团公司与日本本田技研工业株式会社共同出资组建的合资公司,双方各占50%股份,合作年限为30年
宝马这个牌子的汽车怎么样
Intent: car_is_how
Rule: (.*?)(?:这个牌子的汽车|这个汽车品牌|汽车)?(?:怎么样|好用吗|如何呢)
SPARQL:
PREFIX : <http://kgdemo.com/>
SELECT DISTINCT ?o WHERE {
:宝马 :is_how ?o .
}
Output: 德系大品牌值得信赖,各方面口碑都很好
宾利这个牌子的汽车怎么样
Intent: car_is_how
Rule: (.*?)(?:这个牌子的汽车|这个汽车品牌|汽车)?(?:怎么样|好用吗|如何呢)
SPARQL:
PREFIX : <http://kgdemo.com/>
SELECT DISTINCT ?o WHERE {
:宾利 :is_how ?o .
}
Output: 举世闻名的豪华汽车制造品牌,非常昂贵哦
北京奔驰怎么样
Intent: car_is_how
Rule: (.*?)(?:这个牌子的汽车|这个汽车品牌|汽车)?(?:怎么样|好用吗|如何呢)
SPARQL:
PREFIX : <http://kgdemo.com/>
SELECT DISTINCT ?o WHERE {
:北京奔驰 :is_how ?o .
}
Output: 大品牌值得信赖,我经常在宝马的后视镜里看到它
宝马如何呢
Intent: car_is_how
Rule: (.*?)(?:这个牌子的汽车|这个汽车品牌|汽车)?(?:怎么样|好用吗|如何呢)
SPARQL:
PREFIX : <http://kgdemo.com/>
SELECT DISTINCT ?o WHERE {
:宝马 :is_how ?o .
}
Output: 德系大品牌值得信赖,各方面口碑都很好
宝马汽车好用吗
Intent: car_is_how
Rule: (.*?)(?:这个牌子的汽车|这个汽车品牌|汽车)?(?:怎么样|好用吗|如何呢)
SPARQL:
PREFIX : <http://kgdemo.com/>
SELECT DISTINCT ?o WHERE {
:宝马 :is_how ?o .
}
Output: 德系大品牌值得信赖,各方面口碑都很好
宝马和奔驰比怎么样
Intent: car_is_compared
Rule: (.*)和.*比(?:怎么样|哪个好|哪个更好)
SPARQL:
PREFIX : <http://kgdemo.com/>
SELECT DISTINCT ?o WHERE {
:宝马 :is_compared ?o .
}
Output: 各有千秋,但是人生苦短,我选宝马
宝马和奔驰比哪个好
Intent: car_is_compared
Rule: (.*)和.*比(?:怎么样|哪个好|哪个更好)
SPARQL:
PREFIX : <http://kgdemo.com/>
SELECT DISTINCT ?o WHERE {
:宝马 :is_compared ?o .
}
Output: 各有千秋,但是人生苦短,我选宝马
宝马和奔驰比哪个更好
Intent: car_is_compared
Rule: (.*)和.*比(?:怎么样|哪个好|哪个更好)
SPARQL:
PREFIX : <http://kgdemo.com/>
SELECT DISTINCT ?o WHERE {
:宝马 :is_compared ?o .
}
Output: 各有千秋,但是人生苦短,我选宝马
|
_posts/ithome/2020-12th-ironman/7.非監督式學習-降維(1)/t-sne_xgb.ipynb | ###Markdown
載入digits資料集1. 首先我們載入keras所提供的mnist datasets2. 將28*28像素的照片轉換成一維3. 將所有資料正規化(除以255)
###Code
# load data
(X_train, y_train), (X_test, y_test) = mnist.load_data()
X_train=X_train.reshape(len(X_train),-1)/255
X_test=X_test.reshape(len(X_test),-1)/255
X_train
print('Training data shape:',X_train.shape)
print('Testing data shape:',X_test.shape)
plt.imshow(X_train[773].reshape(28,28))
###Output
_____no_output_____
###Markdown
t-SNE降維- n_components: 降維之後的維度- perplexity: 最佳化過程中考慮鄰近點的多寡,default 30,原始paper建議5-50- n_iter: 迭代次數,預設1000
###Code
tsneModel = TSNE(n_components=2, random_state=42,n_iter=2000)
train_reduced = tsneModel.fit_transform(X_train)
plt.figure(figsize=(8,6))
plt.scatter(train_reduced[:, 0], train_reduced[:, 1], c=y_train, alpha=0.5,
cmap=plt.cm.get_cmap('nipy_spectral', 10))
plt.colorbar()
plt.show()
###Output
_____no_output_____
###Markdown
XGBoost(regression) Fit t-SNE model這一步驟是學出一個模型可以直接將784維的(input)資料預測t-sne過後的2D資料。
###Code
from sklearn.multioutput import MultiOutputRegressor
import xgboost
xgb = xgboost.XGBRegressor(colsample_bytree=0.4,
gamma=0,
learning_rate=0.09,
max_depth=6,
min_child_weight=1.5,
n_estimators=5000,
reg_alpha=0.75,
reg_lambda=0.45,
subsample=0.6,
seed=42,
# objective ='reg:squarederror',
predictor='cpu_predictor',
tree_method='gpu_hist')
# xgb = xgboost.XGBRegressor()
xgbModel=MultiOutputRegressor(xgb)
xgbModel.fit(X_train, train_reduced)
trainPred=xgbModel.predict(X_train)
###Output
[21:30:19] WARNING: /workspace/src/objective/regression_obj.cu:152: reg:linear is now deprecated in favor of reg:squarederror.
[21:31:50] WARNING: /workspace/src/objective/regression_obj.cu:152: reg:linear is now deprecated in favor of reg:squarederror.
###Markdown
內部測試
###Code
from sklearn.metrics import mean_squared_error
from sklearn.metrics import mean_absolute_error
from math import sqrt
print("Score: ",xgbModel.score(X_train, train_reduced))
print("MAE: ",mean_absolute_error(trainPred,train_reduced))
print("MSE: ",(mean_squared_error(trainPred,train_reduced)))
print("RMSE: ",sqrt(mean_squared_error(trainPred,train_reduced)))
plt.figure(figsize=(8,6))
plt.scatter(trainPred[:, 0], trainPred[:, 1], c=y_train, alpha=0.5,
cmap=plt.cm.get_cmap('nipy_spectral', 10))
plt.colorbar()
plt.show()
###Output
_____no_output_____
###Markdown
外部測試
###Code
testPred=xgbModel.predict(X_test)
plt.figure(figsize=(8,6))
plt.scatter(testPred[:, 0], testPred[:, 1], c=y_test, alpha=0.5,
cmap=plt.cm.get_cmap('nipy_spectral', 10))
plt.colorbar()
plt.show()
###Output
_____no_output_____
###Markdown
儲存XGBoost(regression) Model
###Code
import pickle
import gzip
# with open('./model/xgb(regression)-42-5000-scale-all.pickle', 'wb') as f:
# pickle.dump(xgbModel, f)
with gzip.GzipFile('./model/xgb(regression)-42-5000-scale-all.pgz', 'w') as f:
pickle.dump(xgbModel, f)
###Output
_____no_output_____
###Markdown
輸出KNN(784D->2D)預測
###Code
X=np.concatenate((X_train, X_test), axis=0)
y=np.concatenate((y_train, y_test), axis=0)
pred=xgbModel.predict(X)
pdData = pd.DataFrame(pred, columns = ["x1", "x2"])
pdData["y"]=y
pdData.to_csv('./model/Result-tsne-42-5000-scale-all(pgz).csv',index=False)
###Output
_____no_output_____ |
0006_teste_hipotese_ANOVA.ipynb | ###Markdown
Análise de variância (ANOVA)- Um gerente suspeita que a desempenho de 3 equipes com diferentes turnos (manhã, tarde, noite) variam. Suspeita que o turno impacte o desempenho da equipe. Dados foram coletados.
###Code
import numpy as np
import pandas as pd
import seaborn as sns
import matplotlib.pyplot as plt
sns.set(color_codes =True)
%matplotlib inline
import statsmodels.api as sm
from scipy import stats
from scipy.stats import ttest_1samp, ttest_ind, mannwhitneyu, levene, shapiro, wilcoxon
AN = pd.read_excel("https://github.com/julianovale/lean_6_sigma_python/blob/master/anova.xlsx?raw=true")
AN
###Output
_____no_output_____
###Markdown
Preparando o DataFrame
###Code
ANS=AN.stack().rename_axis(('Series','Timing')).reset_index(name="Val")
ANS
sns.boxplot(x=ANS.Timing, y=ANS.Val, width = 0.3)
stats.normaltest(ANS.Val).pvalue # se valor-p > 0.05 aceito H0: a amostra é normal
###Output
_____no_output_____
###Markdown
Importações necessárias
###Code
from statsmodels.formula.api import ols
from statsmodels.stats.anova import anova_lm
y=ANS.Val
x=ANS.Timing
formula='y ~ x'
model = ols(formula, ANS).fit()
aov_table = anova_lm(model)
aov_table
###Output
_____no_output_____ |
Tissue_DNA-FISH/CTP13_SE/20220316-NewPostAnalysis_DNA_after_MERFISH_v2_franklin.ipynb | ###Markdown
Analysis of DNA-MERFISH for CTP11by Pu Zheng2022.02.15analysis for dataset:\\10.245.74.158\Chromatin_NAS_0\20220215-P_brain_CTP11-1000_CTP12_from0208This data is DNA of uncleared MERFISH RNA: \\10.245.74.158\Chromatin_NAS_0\20220208-P_brain_M1_nonclear
###Code
%run "..\..\Startup_py3.py"
sys.path.append(r"..\..\..\..\Documents")
import ImageAnalysis3 as ia
%matplotlib notebook
from ImageAnalysis3 import *
print(os.getpid())
import h5py
from ImageAnalysis3.classes import _allowed_kwds
import ast
###Output
26836
###Markdown
1. Pre-processing info
###Code
fov_param = {'data_folder': r'\\10.245.74.158\Chromatin_NAS_4\20220316-P_brain_CTP11-12-13_from_0304',
'save_folder': r'\\mendel\Mendel_SSD2\Pu_Temp\20220316-P_brain_CTP11-12-13_from_0304',
'experiment_type': 'DNA',
'num_threads': 32,
'correction_folder':r'\\10.245.74.158\Chromatin_NAS_0\Corrections\20210621-Corrections_lumencor_from_60_to_50',
'shared_parameters':{
'single_im_size':[50,2048,2048],
'distance_zxy': [250, 108, 108],
'corr_channels':['750','647','561'],
'num_empty_frames': 0,
'num_buffer_frames':0,
'corr_hot_pixel':True,
'corr_Z_shift':False,
'corr_bleed':True,
'min_num_seeds':5,
'max_num_seeds': 20000,
'spot_seeding_th': 1000,
'normalize_intensity_local':False,
'normalize_intensity_background':False,
'corr_gaussian_highpass':False,
},
}
###Output
_____no_output_____
###Markdown
1.1 define required floders
###Code
save_folder = fov_param['save_folder']
save_filenames = [os.path.join(save_folder, _fl) for _fl in os.listdir(save_folder)
if _fl.split(os.extsep)[-1]=='hdf5']
# extract fov_id
save_fov_ids = [int(os.path.basename(_fl).split('.hdf5')[0].split('_')[-1]) for _fl in save_filenames]
debug = False
print(f"{len(save_filenames)} fovs detected")
segmentation_folder = os.path.join(save_folder, 'Segmentation')
if not os.path.exists(segmentation_folder):
os.makedirs(segmentation_folder)
print(f"Creating segmentation_folder: {segmentation_folder}")
else:
print(f"Use segmentation_folder: {segmentation_folder}")
cand_spot_folder = os.path.join(save_folder, 'CandSpots')
if not os.path.exists(cand_spot_folder):
os.makedirs(cand_spot_folder)
print(f"Creating cand_spot_folder: {cand_spot_folder}")
else:
print(f"Use cand_spot_folder: {cand_spot_folder}")
decoder_folder = cand_spot_folder.replace('CandSpots', 'Decoder')
if debug:
_version = 0
while os.path.exists(os.path.join(decoder_folder, f'v{_version}')):
_version += 1
decoder_folder = os.path.join(decoder_folder, f'v{_version}')
if not os.path.exists(decoder_folder):
os.makedirs(decoder_folder)
print(f"Creating decoder_folder: {decoder_folder}")
else:
print(f"Use decoder_folder: {decoder_folder}")
pixel_sizes = np.array(fov_param['shared_parameters']['distance_zxy'])
single_im_size = np.array(fov_param['shared_parameters']['single_im_size'])
intensity_th = np.array(fov_param['shared_parameters']['spot_seeding_th'])
save_fov_ids = save_fov_ids[128:129]
save_filenames = save_filenames[128:129]
###Output
_____no_output_____
###Markdown
2. Translate segmentation 2.1 load rotation matrix
###Code
rna_data_folder
fov_param['data_folder']
# MERFISH segmentation
merfish_segmentation_folder = r'\\mendel\Mendel_SSD3\MERFISH_Analysis\20220304-P_brain_M1_nonclear_adaptors\CellPoseSegment\features'
merfish_dapi_folder = r'\\10.245.74.158\Chromatin_NAS_0\20220304-P_brain_M1_nonclear_adaptors\Segmentation_Cellpose'
if not os.path.exists(merfish_dapi_folder):
os.makedirs(merfish_dapi_folder)
# generate alignment
rna_data_folder = r'\\10.245.74.158\Chromatin_NAS_0\20220304-P_brain_M1_nonclear_adaptors'
rna_alignment_file = os.path.join(rna_data_folder, 'Alignment', '10x_positions_before.txt')
dna_alignment_file = os.path.join(fov_param['data_folder'], 'Alignment', '10x_positions_after.txt')
print(rna_alignment_file, '\n', dna_alignment_file)
print(os.path.exists(rna_alignment_file), os.path.exists(dna_alignment_file))
R, t = ia.correction_tools.alignment.align_manual_points(rna_alignment_file, dna_alignment_file,
save_folder=save_folder)
###Output
\\10.245.74.158\Chromatin_NAS_0\20220304-P_brain_M1_nonclear_adaptors\Alignment\10x_positions_before.txt
\\10.245.74.158\Chromatin_NAS_4\20220316-P_brain_CTP11-12-13_from_0304\Alignment\10x_positions_after.txt
True True
- Manually picked points aligned, rotation:
[[ 0.99997433 -0.00716481]
[ 0.00716481 0.99997433]],
translation:[-238.54348979 -722.53301093]
-- rotation matrix saved to file:\\mendel\Mendel_SSD2\Pu_Temp\20220316-P_brain_CTP11-12-13_from_0304\rotation
-- translation matrix saved to file:\\mendel\Mendel_SSD2\Pu_Temp\20220316-P_brain_CTP11-12-13_from_0304\translation
###Markdown
2.2 save DAPI image for RNA
###Code
from tqdm import tqdm
rna_fds, rna_fovs = ia.io_tools.data.get_folders(rna_data_folder)
ref_fd = rna_fds[0]
overwrite_dapi = False
for _fov_id in tqdm(save_fov_ids):
_dapi_savefile = os.path.join(merfish_dapi_folder, rna_fovs[_fov_id].replace('.dax', '_Dapi.npy'))
if overwrite_dapi or not os.path.exists(_dapi_savefile):
# load
_im = ia.visual_tools.DaxReader(os.path.join(ref_fd, rna_fovs[_fov_id])).loadAll()
_dapi_im = _im[4::5]
# save
np.save(_dapi_savefile.split('.npy')[0], _dapi_im)
###Output
100%|████████████████████████████████████████████████████████████████████████████| 115/115 [00:00<00:00, 677.44it/s]
###Markdown
2.3 Prepare args
###Code
import multiprocessing as mp
# savefile for segmentations
_total_seg_save_file = os.path.join(segmentation_folder, 'full_segmentation.hdf5')
# required parameters
microscope_file = r'\\mendel\pu_documents\Merfish_analysis\Merfish_Analysis_Scripts\merlin_parameters\microscope\storm6_microscope.json'
Zcoords = np.arange(0,12.5,0.25) # z-coordinates of all z-planes in this experiment
seg_align_params = {}
overwrite_segmentation = False
plot_segmentation = True
%%time
# initiate locks
_manager = mp.Manager()
# savefile lock
_segmentation_savefile_lock = _manager.RLock()
_seg_align_args = []
# prepare kwargs
for _fov_id, _save_filename in zip(save_fov_ids, save_filenames):
# segmentation filename
_segmentation_filename = os.path.join(segmentation_folder,
os.path.basename(_save_filename).replace('.hdf5', '_Segmentation.npy') )
_rna_feature_filename = os.path.join(merfish_segmentation_folder, f"feature_data_{_fov_id}.hdf5")
_rna_dapi_filename = os.path.join(merfish_dapi_folder,
os.path.basename(_save_filename).replace('.hdf5', '_Dapi.npy'))
_args = (_fov_id, Zcoords, _rna_feature_filename, _rna_dapi_filename,
_save_filename, microscope_file, R,
_total_seg_save_file, True, _segmentation_savefile_lock,
seg_align_params, plot_segmentation, overwrite_segmentation, False, False, True,
)
_seg_align_args.append(_args)
print(len(_seg_align_args))
###Output
115
Wall time: 207 ms
###Markdown
2.4 test run and plot
###Code
import ImageAnalysis3.segmentation_tools.cell
_seg_cls = ia.segmentation_tools.cell.Align_Segmentation(
_seg_align_args[0][2],_seg_align_args[0][3],_seg_align_args[0][4],
microscope_file, R, debug=True,
)
_dna_mask, _full_rna_mask, _rna_dapi, _rot_rna_dapi, _dna_dapi = _seg_cls._generate_dna_mask(_seg_align_args[0][1])
%matplotlib notebook
plt.style.use('dark_background')
_vis = ia.visual_tools.imshow_mark_3d_v2([_dna_dapi], min_max_default=[10000,50000], image_names=['DNA DAPI'])
_vis.f.savefig(os.path.join(segmentation_folder, 'test_dna_dapi.png'), transparent=True)
%matplotlib notebook
plt.style.use('dark_background')
_vis = ia.visual_tools.imshow_mark_3d_v2([_rna_dapi], min_max_default=[500,8000], image_names=['RNA DAPI'])
_vis.f.savefig(os.path.join(segmentation_folder, 'test_rna_dapi.png'), transparent=True)
###Output
_____no_output_____
###Markdown
2.5 batch run translation
###Code
%%time
from ImageAnalysis3.segmentation_tools.cell import _batch_align_segmentation
# Multiprocessing
print(f"- Start multiprocessing segmentation alignment", end=' ')
_start_time = time.time()
with mp.Pool(16) as _seg_pool:
# start multiprocessing
_seg_pool.starmap(_batch_align_segmentation, _seg_align_args, chunksize=1)
# close multiprocessing
_seg_pool.close()
_seg_pool.join()
_seg_pool.terminate()
print(f"finish in {time.time()-_start_time:.3f}s. ")
###Output
- Start multiprocessing segmentation alignment finish in 417.439s.
Wall time: 7min 9s
###Markdown
non-parallel versionreload(ia.segmentation_tools.cell)reload(correction_tools.alignment)from ImageAnalysis3.segmentation_tools.cell import _batch_align_segmentationfor _args in _seg_align_args: _batch_align_segmentation(*_args)
###Code
# 3 Partition DNA-MERFISH spots
###Output
_____no_output_____
###Markdown
from ImageAnalysis3.classes.partition_spots import Spots_Partitionfrom ImageAnalysis3.classes.preprocess import Spots3Dfrom ImageAnalysis3.figure_tools import plot_partitionimport pandas as pd from ImageAnalysis3.segmentation_tools.cell import Align_Segmentationimport ImageAnalysis3.io_tools.spots reload(segmentation_tools.cell)reload(io_tools.spots)reload(ia.classes.preprocess)reload(ia.classes.partition_spots)
###Code
## 3.1 prepare spots for partition
###Output
_____no_output_____
###Markdown
search_radius = 3overwrite_cand_spots = Falseadd_relabel_spots = True_total_seg_save_file defined in 2.3_partition_args = [] %%timefor _fov_id, _save_filename in zip(save_fov_ids[-1:], save_filenames[-1:]): savename _cand_spot_filename = os.path.join(cand_spot_folder, os.path.basename(_save_filename).replace('.hdf5', f'_CandSpots.csv') ) load segmentation label matrix and uids _align_seg = Align_Segmentation('', '', _save_filename, '', np.array([])) _align_seg._load(_total_seg_save_file) seg_label, fovcell_2_uid = _align_seg.dna_mask, _align_seg.fovcell_2_uid load spots spots_list, combo_bits = ia.io_tools.spots.load_preprocess_spots( _save_filename, 'combo', None, pixel_sizes=pixel_sizes, ) add relabeled spots if specified. if add_relabel_spots: relabel_spots_list, relabel_bits = ia.io_tools.spots.load_preprocess_spots( _save_filename, 'relabeled_combo', None, pixel_sizes=pixel_sizes, ) for _rspots, _rbit in zip(relabel_spots_list, relabel_bits): _ind = list(combo_bits).index(_rbit) _old_spots = spots_list[_ind] merge _merged_spots = ia.io_tools.spots.merge_RelabelSpots( _old_spots, _rspots, pixel_sizes=pixel_sizes, ) replace spots_list[_ind] = _merged_spots _all_spots = ia.io_tools.spots.merge_Spots3DList(spots_list, pixel_sizes=pixel_sizes) partition args _args = ( _fov_id, np.array(_all_spots), _all_spots.bits, _all_spots.channels, seg_label, fovcell_2_uid, search_radius, pixel_sizes, True, True, _cand_spot_filename, True, 60, False, True, ) _partition_args.append(_args) print(len(_partition_args)) %%time Multiprocessingprint(f"- Start multiprocessing spot partitioning", end=' ')_start_time = time.time()with mp.Pool(16) as _partition_pool: start multiprocessing _partition_pool.starmap(ia.classes.partition_spots.batch_partition_DNA_spots, _partition_args, chunksize=1) close multiprocessing _partition_pool.close() _partition_pool.join() _partition_pool.terminate()print(f"finish in {time.time()-_start_time:.3f}s. ")
###Code
# sequential
for _args in _partition_args:
ia.classes.partition_spots.batch_partition_DNA_spots(*_args)
###Output
_____no_output_____
###Markdown
3. Decoding of DNA-MERFISH
###Code
# load two codebooks
import pandas as pd
codebook_gn = pd.read_csv(r'\\10.245.74.212\Chromatin_NAS_2\Chromatin_Libraries\CTP-11_brain\Summary_tables\CTP11-mouse-genome-1000_codebook.csv', header=0)
codebook_se = pd.read_csv(r'\\10.245.74.212\Chromatin_NAS_2\Chromatin_Libraries\CTP-13_brain-super-enhancers\Summary_tables\CTP13-super-enhancers-1000_codebook.csv', header=0)
codebook_se['id'] = codebook_se['id'] + np.max(codebook_gn['id'])
###Output
_____no_output_____
###Markdown
re-sort chr_order
###Code
# combine region_ids
merged_codebook = pd.concat([codebook_gn, codebook_se], axis=0, join='outer',ignore_index=True).fillna(0)
merged_codebook['reg_start'] = [int(_name.split(':')[1].split('-')[0]) for _name in merged_codebook['name']]
merged_codebook['reg_end'] = [int(_name.split(':')[1].split('-')[1]) for _name in merged_codebook['name']]
merged_codebook['reg_mid'] = (merged_codebook['reg_start'] + merged_codebook['reg_end'])/2
for _chr in np.unique(merged_codebook['chr']):
_chr_codebook = merged_codebook[merged_codebook['chr']==_chr]
_reg_order = np.argsort(merged_codebook.loc[merged_codebook['chr']==_chr, 'reg_mid'])
merged_codebook.loc[_chr_codebook.index[_reg_order], 'chr_order'] = np.arange(len(_chr_codebook))
# cleanup
codebook_df = merged_codebook[[_c for _c in merged_codebook.columns if 'reg_' not in _c]]
codebook_df
codebook_df.to_csv(os.path.join(decoder_folder, 'merged_codebook.csv'), index=False)
###Output
_____no_output_____
###Markdown
3.2 load spot files
###Code
with h5py.File(save_filenames[0], "r", libver='latest') as _f:
_grp = _f['combo']
combo_channels = [_ch.decode() for _ch in _grp['channels'][:]]
combo_ids = _grp['ids'][:]
bit_2_channel = {_b:_ch for _b,_ch in zip(combo_ids, combo_channels)}
###Output
_____no_output_____
###Markdown
3.3 prepare decoding args
###Code
from ImageAnalysis3.classes import decode
reload(decode)
%%time
from tqdm import tqdm
from ImageAnalysis3.classes import decode
reload(decode)
overwrite_decoder = False
return_decoder = False
skip_exist = True
load_exist = True
pair_search_radius = 300
decode_args = []
for _fov_id, _save_filename in zip(save_fov_ids, save_filenames):
print(f"- Preparing decoding args for fov: {_fov_id}")
# load candidate spots for the fov
cand_spot_filename = os.path.join(cand_spot_folder,
os.path.basename(_save_filename).replace('.hdf5', f'_CandSpots.csv') )
if os.path.isfile(cand_spot_filename):
_fov_spots_df = pd.read_csv(cand_spot_filename)
else:
continue # skip if fov doesn't exist
for _cell_id in np.unique(_fov_spots_df['cell_id']):
# get decoder filename
_decoder_filename = os.path.join(decoder_folder, f'Fov-{_fov_id}_Cell-{_cell_id}_Decoder.hdf5')
if os.path.exists(_decoder_filename) and skip_exist:
continue
# get cell_df
_cell_spots_df =_fov_spots_df[_fov_spots_df['cell_id']==_cell_id]
_args = (_cell_spots_df, codebook_df, _decoder_filename, combo_ids,
False, True, bit_2_channel,
pixel_sizes, 2, 0.1,
pair_search_radius, -1, 1, 5, 0, -25,
load_exist, overwrite_decoder, return_decoder, False)
# append
decode_args.append(_args)
print(len(decode_args))
###Output
- Preparing decoding args for fov: 128
13
Wall time: 4.8 s
###Markdown
3.4 test decode one cell
###Code
# test run one cell
%matplotlib inline
reload(decode)
_cell_ind = 30
test_arg = list(decode_args[_cell_ind])
test_arg[16] = True
test_arg[18] = True
decoder = decode.batch_decode_DNA(*tuple(test_arg))
###Output
Not enough cand_spots (960) found, skip.
###Markdown
3.4.2 visualize decoded spots
###Code
if not os.path.exists(decoder_folder):
os.makedirs(decoder_folder)
print(f"Creating decoder_folder: {decoder_folder}")
else:
print(f"Use decoder_folder: {decoder_folder}")
decode_figure_folder = os.path.join(decoder_folder, 'Figures')
if not os.path.exists(decode_figure_folder):
os.makedirs(decode_figure_folder)
print(f"Creating decode_figure_folder: {decode_figure_folder}")
else:
print(f"Use decode_figure_folder: {decode_figure_folder}")
%matplotlib notebook
def rotate(angle):
ax.view_init(azim=angle)
from matplotlib import animation
from matplotlib.cm import Reds, Blues, Spectral
fig = plt.figure(dpi=150)
ax = fig.add_subplot(projection='3d')
_chr_name = '1'
_zxys_list = np.array(decoder.chr_2_zxys_list[_chr_name])/1000
for _ichr, _zxys in enumerate(_zxys_list):
ax.scatter(_zxys[:,1], _zxys[:,2], _zxys[:,0],
cmap=Spectral,
c=Spectral(_ichr/(len(_zxys_list)+1)),
alpha=0.7,
s=3)
ax.plot(_zxys[:,1], _zxys[:,2], _zxys[:,0], linewidth=0.5,
alpha=0.7,
color = Spectral( _ichr/(len(_zxys_list)+1) ) )
ax.grid(False)
ax.xaxis.set_pane_color((0.0, 0.0, 0.0, 0.0))
ax.yaxis.set_pane_color((0.0, 0.0, 0.0, 0.0))
ax.zaxis.set_pane_color((0.0, 0.0, 0.0, 0.0))
angle = 2
ani = animation.FuncAnimation(fig, rotate, frames=np.arange(0, 360, angle), interval=30)
ani.save(os.path.join(decode_figure_folder,
os.path.basename(decoder.savefile).replace('.hdf5', f'_Picked_chr-{_chr_name}.gif')),
writer=animation.PillowWriter(fps=30))
plt.show()
###Output
_____no_output_____
###Markdown
3.5 process all
###Code
%%time
# old version
import multiprocessing as mp
print(len(decode_args))
with mp.Pool(20) as decode_pool:
decode_results = decode_pool.starmap(decode.batch_decode_DNA, decode_args, chunksize=1)
decode_pool.close()
decode_pool.join()
decode_pool.terminate()
###Output
13
Wall time: 1min 5s
|
knn-version/[start_here] 1_encode_faces.ipynb | ###Markdown
Face Recognition in Low ResolutionThis file is for step 1: face encoding.Be sure to run all code blocks. Click the code blocks, then use `CTRL`+`Enter` to run. Be sure you run them in sequence Step 1: Encode faces Purpose of this code is to quantify faces inside training set. We will be using a network that is already trained to create 128-d embeddings. Step 1.1: Imports & Path Setup
###Code
# import required packages to run
from imutils import paths # to get paths
import cv2 # for face detection
import os # for file system access
import pickle # for storing embeddings
import face_recognition # for embeddings
from matplotlib import pyplot as plt
datasetPathAbs = os.path.abspath(args["dataset"])
if not os.path.exists(args["dataset"]):
print("[WARN] ", datasetPathAbs, "folder does not exist!")
print("Create a folder there, and put subfolders named as the person's name (e.g. 'Long_Shun') and put images inside!")
args["start_check"] = "false"
elif not os.access('.', os.W_OK):
print("[ERROR] Insufficient permission to write to current directory. You might want to check write permissions of the folder.")
args["start_check"] = "false"
else:
print("[INFO] Imports & Path set successfully!")
args["start_check"] = "true"
###Output
[INFO] Imports & Path set successfully!
###Markdown
Step 1.2: Detect & encode facesDetect face, encode them into the system, and then store the encodings inside the .pickle file
###Code
# set arguments
args = {}
args["dataset"] = "dataset\dataset-full-res"
args["detection_method"] = "hog" # choose between 'hog', 'cnn'
args["encodings"] = "encodings.pickle"
args["start_check"] = "false"
print("[NOTE] Arguments set successfully")
# get input image paths
print("[NOTE] Quantifying Faces")
imagePaths = list(paths.list_images(args["dataset"]))
if not imagePaths:
print("[ERROR] No image found in '", datasetPathAbs, "' did you forgot to put the images in the dataset folder?")
else:
# initialize the list of known encodings and known names
knownEncodings = []
knownNames = []
# FOR DEBUGGING
#print(imagePaths)
# loop over the image paths
for (i, imagePath) in enumerate(imagePaths):
# extract the person name from the image path
print("[INFO] processing image [{}/{}]".format(i + 1, len(imagePaths)), imagePath)
name = imagePath.split(os.path.sep)[-2]
# load the input image and convert it from RGB (OpenCV ordering)
# to dlib ordering (RGB)
image = cv2.imread(imagePath)
rgb = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
# detect the (x, y)-coordinates of the bounding boxes
# corresponding to each face in the input image
boxes = face_recognition.face_locations(rgb, model=args["detection_method"])
print(len(boxes))
if len(boxes) == 0:
print("[WARN] Can't find faces! ", imagePath)
print("This image will be excluded from encoding, replace/delete the image to remove this warning.")
elif len(boxes) > 1:
print("[WARN] Multiple faces found! ", imagePath)
print("This image will be excluded from encoding, replace/delete the image to remove this warning")
else:
# compute the facial embedding for the face
encodings = face_recognition.face_encodings(rgb, boxes)
# loop over the encodings
for encoding in encodings:
# add each encoding + name to our set of known names and
# encodings
knownEncodings.append(encoding)
knownNames.append(name)
for (top, right, bottom, left) in boxes:
# draw the predicted face name on the image
cv2.rectangle(rgb, (left, top), (right, bottom), (255, 0, 0), 1)
# error checks
plt.imshow(rgb)
plt.title(imagePath)
plt.show()
# dump the facial encodings + names to disk
print("[INFO] serializing encodings...")
data = {"encodings": knownEncodings, "names": knownNames}
f = open(args["encodings"], "wb")
f.write(pickle.dumps(data))
f.close()
print("[INFO] Serialization Complete!")
# remove duplicates from list
dedupedNames = []
[dedupedNames.append(x) for x in knownNames if x not in dedupedNames]
print("Serialized people: ", len(dedupedNames))
print("Serialized names: ", dedupedNames)
print("You can now open 2_recognize_faces_in_image Jupyter Notebook to detect the faces of the above person!")
# from fdet import MTCNN
# detector = MTCNN()
# # get input image paths
# print("[NOTE] Quantifying Faces")
# imagePaths = list(paths.list_images(args["dataset"]))
# # initialize the list of known encodings and known names
# knownEncodings = []
# knownNames = []
# # FOR DEBUGGING
# #print(imagePaths)
# # loop over the image paths
# for (i, imagePath) in enumerate(imagePaths):
# # extract the person name from the image path
# print("[INFO] processing image {}/{} in {}".format(i + 1, len(imagePaths), imagePath))
# name = imagePath.split(os.path.sep)[-2]
# # load the input image and convert it from RGB (OpenCV ordering)
# # to dlib ordering (RGB)
# image = cv2.imread(imagePath)
# rgb = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
# # detect the (x, y)-coordinates of the bounding boxes
# # corresponding to each face in the input image
# boxes = detector.detect(rgb)
# # print(boxes)
# # compute the facial embedding for the face
# # print("[NOTE]", len(boxes), " faces found in ", imagePath)
# for box in boxes:
# boxFormatted = [tuple(box.get('box'))]
# encodings = face_recognition.face_encodings(rgb,boxFormatted)
# # loop over the encodings
# for encoding in encodings:
# # add each encoding + name to our set of known names and
# # encodings
# knownEncodings.append(encoding)
# knownNames.append(name)
# # dump the facial encodings + names to disk
# print("[INFO] serializing encodings...")
# data = {"encodings": knownEncodings, "names": knownNames}
# f = open(args["encodings"], "wb")
# f.write(pickle.dumps(data))
# f.close()
# print("[INFO] Complete!")
###Output
_____no_output_____ |
EXL_EQ_2022.ipynb | ###Markdown
Importing the library
###Code
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
%matplotlib inline
###Output
_____no_output_____
###Markdown
Reading the excel sheet
###Code
df = pd.read_excel('Historical_data.xlsx')
df2 = df.copy()
print(pd.crosstab(df['personal_loan'],df['term_deposit_subscribed']))
df.head()
###Output
_____no_output_____
###Markdown
Checking for unwanted columns There is no unwanted column Checking for columns with single value There is no column with single value Finding the missing values in different columns
###Code
df.isnull().sum()
###Output
_____no_output_____
###Markdown
Replacing the NaN age column by ``mean`` age & NaN personal loan ``no``
###Code
df['customer_age'] = df['customer_age'].replace(np.NaN,int(df['customer_age'].mean()))
df['personal_loan'] = df['personal_loan'].replace(np.NaN,'no')
df.isnull().sum()
###Output
_____no_output_____
###Markdown
Printing the index of missing values of `balance` column and `subscription` status
###Code
balance = df['balance'].values.reshape(len(df),1)
result = df['term_deposit_subscribed'].values.reshape(len(df),1)
for item in np.argwhere(np.isnan(np.concatenate((balance,result),axis=1))):
print(item)
###Output
[42 0]
[88 0]
[398 0]
[518 0]
[1037 0]
[1051 0]
[1057 0]
[1112 0]
[1162 0]
[1246 0]
[1313 0]
[1330 0]
[1571 0]
[1781 0]
[1814 0]
[1820 0]
[1824 0]
[1853 0]
[2009 0]
[2082 0]
[2206 0]
[2256 0]
[2537 0]
[2553 0]
[2623 0]
[2632 0]
[2652 0]
[2715 0]
[2766 0]
[2813 0]
[2940 0]
[3191 0]
[3224 0]
[3258 0]
[3453 0]
[3558 0]
[3752 0]
[3889 0]
[4073 0]
[4466 0]
[4546 0]
[4562 0]
[4724 0]
[4780 0]
[4800 0]
[4804 0]
[4999 0]
[5021 0]
[5034 0]
[5046 0]
[5084 0]
[5092 0]
[5226 0]
[5236 0]
[5361 0]
[5668 0]
[5863 0]
[5870 0]
[6059 0]
[6078 0]
[6106 0]
[6130 0]
[6147 0]
[6526 0]
[6542 0]
[6740 0]
[6881 0]
[6981 0]
[6992 0]
[7138 0]
[7156 0]
[7164 0]
[7566 0]
[7751 0]
[7766 0]
[7800 0]
[7844 0]
[7993 0]
[7995 0]
[8056 0]
[8144 0]
[8208 0]
[8385 0]
[8606 0]
[8732 0]
[8843 0]
[8943 0]
[8951 0]
[9274 0]
[9379 0]
[9440 0]
[9461 0]
[9487 0]
[9503 0]
[9577 0]
[9666 0]
[9779 0]
[9784 0]
[10043 0]
[10088 0]
[10127 0]
[10146 0]
[10149 0]
[10235 0]
[10247 0]
[10267 0]
[10306 0]
[10333 0]
[10357 0]
[10439 0]
[10568 0]
[10580 0]
[10604 0]
[10653 0]
[10662 0]
[10683 0]
[10708 0]
[10738 0]
[10757 0]
[10814 0]
[10847 0]
[10971 0]
[11107 0]
[11139 0]
[11444 0]
[11495 0]
[11515 0]
[11577 0]
[11720 0]
[11981 0]
[12057 0]
[12064 0]
[12067 0]
[12125 0]
[12171 0]
[12402 0]
[12448 0]
[12487 0]
[12488 0]
[12520 0]
[12584 0]
[12650 0]
[12730 0]
[12820 0]
[12909 0]
[12957 0]
[13183 0]
[13296 0]
[13540 0]
[13578 0]
[13671 0]
[14016 0]
[14300 0]
[14353 0]
[14417 0]
[14521 0]
[14540 0]
[14545 0]
[14575 0]
[14663 0]
[14853 0]
[14908 0]
[15073 0]
[15122 0]
[15155 0]
[15373 0]
[15494 0]
[15589 0]
[15624 0]
[15647 0]
[15652 0]
[15746 0]
[15796 0]
[15865 0]
[15875 0]
[15886 0]
[15899 0]
[15932 0]
[15975 0]
[16112 0]
[16212 0]
[16319 0]
[16333 0]
[16465 0]
[16590 0]
[16633 0]
[16680 0]
[16690 0]
[16860 0]
[17063 0]
[17113 0]
[17122 0]
[17129 0]
[17159 0]
[17164 0]
[17283 0]
[17388 0]
[17565 0]
[17647 0]
[17654 0]
[17713 0]
[18024 0]
[18135 0]
[18171 0]
[18180 0]
[18229 0]
[18291 0]
[18318 0]
[18333 0]
[18550 0]
[18701 0]
[18720 0]
[18727 0]
[18730 0]
[18874 0]
[19003 0]
[19113 0]
[19156 0]
[19293 0]
[19525 0]
[19534 0]
[19673 0]
[19686 0]
[19704 0]
[19736 0]
[19830 0]
[19867 0]
[19882 0]
[19935 0]
[19950 0]
[20072 0]
[20156 0]
[20162 0]
[20342 0]
[20379 0]
[20448 0]
[20460 0]
[20661 0]
[20883 0]
[20907 0]
[20936 0]
[21051 0]
[21059 0]
[21163 0]
[21262 0]
[21288 0]
[21303 0]
[21506 0]
[21533 0]
[21570 0]
[21858 0]
[21919 0]
[21982 0]
[22024 0]
[22078 0]
[22302 0]
[22305 0]
[22602 0]
[22630 0]
[22785 0]
[22789 0]
[22848 0]
[23075 0]
[23128 0]
[23165 0]
[23166 0]
[23195 0]
[23239 0]
[23282 0]
[23325 0]
[23496 0]
[23548 0]
[23552 0]
[23598 0]
[23628 0]
[23646 0]
[23778 0]
###Markdown
Replacing the missing values of recent campaign contacts with previous campaign contacts
###Code
num = df['num_contacts_in_campaign'].values.reshape(len(df),1) # To get rid of the missing values present in balance column,
prev = df['num_contacts_prev_campaign'].values.reshape(len(df),1) # we replaced them with values of previous campaign
index = []
for i in np.argwhere(np.isnan(np.concatenate((num,prev),axis=1))):
index.append(i[0])
con = np.concatenate((num,prev),axis=1)
for i in index:
con[i][0] = con[i][1]
# print(con[i])
dic = {}
for i in range(0,len(index)):
dic[index[i]] = con[i][0]
print(dic)
n = df['num_contacts_in_campaign'].values
for i in range(0,len(n)):
if i in dic:
n[i] = dic[i]
df['num_contacts_in_campaign'] = pd.Series(n)
df = df.dropna()
df3 = df.copy()
df3.head()
df.columns
count = 0
for item in df3['balance'].values:
if item < 0 :
count = count +1
print(count)
i = 0
for item in zip(df3['balance'].values,df3['term_deposit_subscribed'].values):
if i < 2960:
if item[1] == 1 and item[0] < 0:
print(item)
i = i+1
if i == 2960:
break
for item in df3.columns[2:]:
if item not in ['balance','communication_type','month',
'last_contact_duration','num_contacts_in_campaign','num_contacts_prev_campaign'] :
print(df3[item].value_counts())
print()
for item in df3.columns[2:]:
if item not in ['default','balance','communication_type',
'last_contact_duration','num_contacts_in_campaign',
'num_contacts_prev_campaign','term_deposit_subscribed'] :
print(pd.crosstab(df3[item],df3['term_deposit_subscribed']))
print()
df3.head()
df3 = df3.iloc[:,1:]
df3.head()
###Output
_____no_output_____
###Markdown
Exploring the categorical features
###Code
categorical_features = [feature for feature in df3.columns if ((df3[feature].dtypes=='O') & (feature not in ['term_deposit_subscribed']))]
categorical_features
for feature in categorical_features:
print("The feature {} and number of categories are {}".format(feature,len(df3[feature].unique())))
###Output
The feature job_type and number of categories are 12
The feature marital and number of categories are 3
The feature education and number of categories are 4
The feature default and number of categories are 2
The feature housing_loan and number of categories are 2
The feature personal_loan and number of categories are 2
The feature communication_type and number of categories are 3
The feature month and number of categories are 12
The feature prev_campaign_outcome and number of categories are 4
###Markdown
InferenceThere are 9 categorical featuresFeatures job type and month have highest number of categorical features Categorical feature distribution
###Code
plt.figure (figsize=(15,80), facecolor ='white') # Now we will plot barplots of the categorical feature
plotnumber =1 # distribution to get a rough idea of different ratios
for categorical_feature in categorical_features:
ax = plt.subplot (12,3, plotnumber)
sns.countplot (y = categorical_feature, data=df3)
plt.xlabel (categorical_feature)
plt.title(categorical_feature)
plotnumber += 1
plt.show()
###Output
_____no_output_____
###Markdown
Inference➤For clients with job type as blue collar, records are highest while for students it is lowest➤For clients with marital status married, records are highest while for divorced it is lowest➤For clients with education qualification secondary, records are highest while for primary it is lowest➤Default doesn't seem to play important role since the yes to no ratio is very high, so we can drop it➤Data in month of May is highest, while in December it is lowest Relationship between categorical features and labels
###Code
for categorical_feature in categorical_features:
sns.catplot(x='term_deposit_subscribed',col=categorical_feature,kind='count',data=df3)
plt.show()
for categorical_feature in categorical_features:
print(df3.groupby(['term_deposit_subscribed',categorical_feature]).size())
###Output
term_deposit_subscribed job_type
0 admin. 2394
blue-collar 4751
entrepreneur 700
housemaid 599
management 4328
retired 897
self-employed 736
services 2013
student 357
technician 3522
unemployed 561
unknown 135
1 admin. 276
blue-collar 330
entrepreneur 62
housemaid 48
management 605
retired 253
self-employed 100
services 182
student 137
technician 392
unemployed 103
unknown 19
dtype: int64
term_deposit_subscribed marital
0 divorced 2476
married 12789
single 5728
1 divorced 291
married 1309
single 907
dtype: int64
term_deposit_subscribed education
0 primary 3237
secondary 10969
tertiary 5938
unknown 849
1 primary 279
secondary 1155
tertiary 953
unknown 120
dtype: int64
term_deposit_subscribed default
0 no 20598
yes 395
1 no 2481
yes 26
dtype: int64
term_deposit_subscribed housing_loan
0 no 8734
yes 12259
1 no 1611
yes 896
dtype: int64
term_deposit_subscribed personal_loan
0 no 17502
yes 3491
1 no 2293
yes 214
dtype: int64
term_deposit_subscribed communication_type
0 cellular 13150
telephone 1284
unknown 6559
1 cellular 2086
telephone 194
unknown 227
dtype: int64
term_deposit_subscribed month
0 apr 1276
aug 2899
dec 62
feb 1150
jan 649
jul 3276
jun 2511
mar 125
may 6783
nov 1885
oct 218
sep 159
1 apr 274
aug 312
dec 48
feb 210
jan 66
jul 276
jun 255
mar 133
may 425
nov 191
oct 164
sep 153
dtype: int64
term_deposit_subscribed prev_campaign_outcome
0 failure 2289
other 790
success 259
unknown 17655
1 failure 286
other 137
success 530
unknown 1554
dtype: int64
###Markdown
Inference➤Students have highest interest on deposit➤Client who has housing loan doesn't seem interested in deposit➤If for a client the previous campaign outcome was a success then there is a high chance of deposit➤In the month of March,September,October,December clients show high interest on deposit➤In May the record is high but the client interest ratio is less Exploring the numerical features
###Code
numerical_features = [feature for feature in df3.columns if ((df3[feature].dtypes != 'O') & (feature not in ['term_deposit_subscribed']))]
print("Number of numerical variable : ",len(numerical_features))
df3[numerical_features].head()
###Output
Number of numerical variable : 6
###Markdown
Inference➤There are 6 numerical features Finding discrete numerical features
###Code
discrete_feature = [feature for feature in numerical_features if len(df3[feature].unique()) < 25]
print("Descrete variables Count : {}".format(len(discrete_feature)))
###Output
Descrete variables Count : 0
###Markdown
Inference➤There is no discrete numerical feature Finding continuous numerical features
###Code
continuous_features = [feature for feature in numerical_features if feature not in discrete_feature+['term_deposit_subscribed']]
print(continuous_features)
print("Continuous feature Count {}".format(len(continuous_features)))
###Output
['customer_age', 'balance', 'day_of_month', 'last_contact_duration', 'num_contacts_in_campaign', 'num_contacts_prev_campaign']
Continuous feature Count 6
###Markdown
Inference➤There are 6 continuous numerical features Continuous numerical feature distribution
###Code
plt.figure(figsize=(20,60),facecolor='white')
plotnumber = 1
for continuous_feature in continuous_features:
ax = plt.subplot(12,3,plotnumber)
sns.distplot(df3[continuous_feature])
plt.xlabel(continuous_feature)
plotnumber += 1
plt.show()
###Output
/usr/local/lib/python3.7/dist-packages/seaborn/distributions.py:2619: FutureWarning: `distplot` is a deprecated function and will be removed in a future version. Please adapt your code to use either `displot` (a figure-level function with similar flexibility) or `histplot` (an axes-level function for histograms).
warnings.warn(msg, FutureWarning)
/usr/local/lib/python3.7/dist-packages/seaborn/distributions.py:2619: FutureWarning: `distplot` is a deprecated function and will be removed in a future version. Please adapt your code to use either `displot` (a figure-level function with similar flexibility) or `histplot` (an axes-level function for histograms).
warnings.warn(msg, FutureWarning)
/usr/local/lib/python3.7/dist-packages/seaborn/distributions.py:2619: FutureWarning: `distplot` is a deprecated function and will be removed in a future version. Please adapt your code to use either `displot` (a figure-level function with similar flexibility) or `histplot` (an axes-level function for histograms).
warnings.warn(msg, FutureWarning)
/usr/local/lib/python3.7/dist-packages/seaborn/distributions.py:2619: FutureWarning: `distplot` is a deprecated function and will be removed in a future version. Please adapt your code to use either `displot` (a figure-level function with similar flexibility) or `histplot` (an axes-level function for histograms).
warnings.warn(msg, FutureWarning)
/usr/local/lib/python3.7/dist-packages/seaborn/distributions.py:2619: FutureWarning: `distplot` is a deprecated function and will be removed in a future version. Please adapt your code to use either `displot` (a figure-level function with similar flexibility) or `histplot` (an axes-level function for histograms).
warnings.warn(msg, FutureWarning)
/usr/local/lib/python3.7/dist-packages/seaborn/distributions.py:2619: FutureWarning: `distplot` is a deprecated function and will be removed in a future version. Please adapt your code to use either `displot` (a figure-level function with similar flexibility) or `histplot` (an axes-level function for histograms).
warnings.warn(msg, FutureWarning)
###Markdown
Inference➤It seems customer age,day of month and last contact duration are distributed normally➤Balance,number of contacts in recent and previous campaign are heavily skewed towards left and seem to have some outliers Relationship between continuous numerical features and labels
###Code
plt.figure(figsize=(20,60),facecolor='white')
plotnumber = 1
for feature in continuous_features:
ax = plt.subplot(12,3,plotnumber)
sns.boxenplot(x="term_deposit_subscribed",y=df3[feature],data = df3)
plt.xlabel(feature)
plotnumber += 1
plt.show()
###Output
_____no_output_____
###Markdown
Finding outliers in numerical features
###Code
plt.figure(figsize=(20,60),facecolor='white')
plotnumber = 1
for numerical_feature in numerical_features:
ax = plt.subplot(12,3,plotnumber)
sns.boxenplot(df3[numerical_feature])
plt.xlabel(numerical_feature)
plotnumber += 1
plt.show()
###Output
/usr/local/lib/python3.7/dist-packages/seaborn/_decorators.py:43: FutureWarning: Pass the following variable as a keyword arg: x. From version 0.12, the only valid positional argument will be `data`, and passing other arguments without an explicit keyword will result in an error or misinterpretation.
FutureWarning
/usr/local/lib/python3.7/dist-packages/seaborn/_decorators.py:43: FutureWarning: Pass the following variable as a keyword arg: x. From version 0.12, the only valid positional argument will be `data`, and passing other arguments without an explicit keyword will result in an error or misinterpretation.
FutureWarning
/usr/local/lib/python3.7/dist-packages/seaborn/_decorators.py:43: FutureWarning: Pass the following variable as a keyword arg: x. From version 0.12, the only valid positional argument will be `data`, and passing other arguments without an explicit keyword will result in an error or misinterpretation.
FutureWarning
/usr/local/lib/python3.7/dist-packages/seaborn/_decorators.py:43: FutureWarning: Pass the following variable as a keyword arg: x. From version 0.12, the only valid positional argument will be `data`, and passing other arguments without an explicit keyword will result in an error or misinterpretation.
FutureWarning
/usr/local/lib/python3.7/dist-packages/seaborn/_decorators.py:43: FutureWarning: Pass the following variable as a keyword arg: x. From version 0.12, the only valid positional argument will be `data`, and passing other arguments without an explicit keyword will result in an error or misinterpretation.
FutureWarning
/usr/local/lib/python3.7/dist-packages/seaborn/_decorators.py:43: FutureWarning: Pass the following variable as a keyword arg: x. From version 0.12, the only valid positional argument will be `data`, and passing other arguments without an explicit keyword will result in an error or misinterpretation.
FutureWarning
###Markdown
Inference➤Customer age,balance,last contact duration,number of contacts in recent and previous campaign have some outliers Exploring the correlationship between numerical features
###Code
cor_mat = df3.corr()
fig = plt.figure(figsize=(15,7))
sns.heatmap(cor_mat,annot=True)
###Output
_____no_output_____
###Markdown
Inference➤No feature is heavily correlated with other features Distribution of customers based on subscription status
###Code
sns.countplot(x="term_deposit_subscribed",data = df3)
plt.show()
df3['term_deposit_subscribed'].groupby(df3['term_deposit_subscribed']).count()
df2 = df3.copy()
df2.head()
df2.shape
###Output
_____no_output_____
###Markdown
Feature Engineering Dropping unwanted features
###Code
df2.groupby(['term_deposit_subscribed','default']).size()
df2.drop(['default'],axis=1,inplace=True)
###Output
_____no_output_____
###Markdown
Removing outliers
###Code
df2.groupby(['term_deposit_subscribed','last_contact_duration'],sort=True)['last_contact_duration'].count()
df2.groupby(['term_deposit_subscribed','num_contacts_in_campaign'],sort=True)['num_contacts_in_campaign'].count()
for item in df2.groupby(['term_deposit_subscribed','num_contacts_in_campaign'],sort=True)['num_contacts_in_campaign']:
[print(i) for i in item]
df2.groupby(['term_deposit_subscribed','num_contacts_prev_campaign'],sort=True)['num_contacts_prev_campaign'].count()
df3 = df2[df2['num_contacts_in_campaign'] < 22]
df3.isnull().sum()
df3.groupby(['term_deposit_subscribed','num_contacts_in_campaign'],sort=True)['num_contacts_in_campaign'].count()
df4 = df3[df3['num_contacts_in_campaign'] < 22]
###Output
_____no_output_____
###Markdown
Converting categorical features into numerical features
###Code
cat_columns = ['job_type','marital','education','communication_type','month','prev_campaign_outcome']
for col in cat_columns:
df4 = pd.concat([df4.drop(col,axis=1),pd.get_dummies(df4[col],prefix=col,prefix_sep="_",drop_first=True,dummy_na=False)],axis=1)
bool_columns = ['housing_loan', 'personal_loan']
for col in bool_columns:
df4[col+'_new']=df4[col].apply(lambda x : 1 if x == 'yes' else 0)
df4.drop(col, axis=1, inplace=True)
df4.head()
df4.columns
###Output
_____no_output_____
###Markdown
Splitting dataset into training set and test set
###Code
y = df4['term_deposit_subscribed'].values
print(y)
df5 = df4.copy()
df5 = df5.drop(['term_deposit_subscribed'],axis=1)
X = df5.iloc[:,:].values
print(len(X))
print(len(y))
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(X,y,test_size=0.2, random_state=0)
###Output
_____no_output_____
###Markdown
Model Selection
###Code
from sklearn.ensemble import RandomForestClassifier
from xgboost import XGBClassifier
from sklearn.model_selection import GridSearchCV
from sklearn.model_selection import cross_val_score
model_score =cross_val_score(estimator=RandomForestClassifier(),X=X_train, y=y_train, cv=5)
print(model_score)
print(model_score.mean())
from sklearn.model_selection import cross_val_score
model_score =cross_val_score(estimator=XGBClassifier(),X=X_train, y=y_train, cv=5)
print(model_score)
print(model_score.mean())
model_param = {
'RandomForestClassifier':{
'model':RandomForestClassifier(),
'param':{
'n_estimators': [10, 50, 100, 130],
'criterion': ['gini', 'entropy'],
'max_depth': range(2, 4, 1),
'max_features': ['auto', 'log2']
}
},
'XGBClassifier':{
'model':XGBClassifier(objective='binary:logistic'),
'param':{
'learning_rate': [0.5, 0.1, 0.01, 0.001],
'max_depth': [3, 5, 10, 20],
'n_estimators': [10, 50, 100, 200]
}
}
}
scores =[]
for model_name, mp in model_param.items():
model_selection = GridSearchCV(estimator=mp['model'],param_grid=mp['param'],cv=5,return_train_score=False)
model_selection.fit(X,y)
scores.append({
'model': model_name,
'best_score': model_selection.best_score_,
'best_params': model_selection.best_params_
})
scores
###Output
_____no_output_____
###Markdown
Building Machine Learning Model **XGB Classifier**
###Code
model_xgb = XGBClassifier(objective='binary:logistic',learning_rate=0.1,max_depth=10,n_estimators=100)
model_xgb.fit(X_train,y_train)
model_xgb.score(X_test,y_test)
headers = ["name", "score"]
values = sorted(zip(df5.columns, model_xgb.feature_importances_), key=lambda x: x[1] * -1)
xgb_feature_importances = pd.DataFrame(values, columns = headers)
#plot feature importances
fig = plt.figure(figsize=(15,7))
x_pos = np.arange(0, len(xgb_feature_importances))
plt.bar(x_pos, xgb_feature_importances['score'])
plt.xticks(x_pos, xgb_feature_importances['name'])
plt.xticks(rotation=90)
plt.title('Feature importances (XGB)')
plt.show()
#Confusion Matrix
from sklearn.metrics import confusion_matrix
cm = confusion_matrix(y_test,model_xgb.predict(X_test))
cm
y_pred = model_xgb.predict(X_test)
for item in np.concatenate((y_test.reshape(len(y_test),1),y_pred.reshape(len(y_pred),1)),1):
print(item)
#plot the graph
from matplotlib import pyplot as plt
import seaborn as sn
sn.heatmap(cm, annot=True)
plt.xlabel('Predicted')
plt.ylabel('True Value')
plt.show()
###Output
_____no_output_____
###Markdown
Scaling the Features
###Code
from sklearn.preprocessing import StandardScaler
sc = StandardScaler()
X_train_scaled = sc.fit_transform(X_train)
X_test_scaled = sc.transform(X_test)
###Output
_____no_output_____
###Markdown
**Logistic Regression**
###Code
from sklearn.linear_model import LogisticRegression
classifier = LogisticRegression(random_state=0)
classifier.fit(X_train_scaled,y_train)
y_pred_log = classifier.predict(X_test_scaled)
from sklearn.metrics import confusion_matrix,accuracy_score
cmlog = confusion_matrix(y_test,y_pred_log)
accuracy = accuracy_score(y_test,y_pred_log)
print(cmlog)
print(accuracy)
###Output
[[4093 66]
[ 408 111]]
0.8986746472851646
###Markdown
**KNN**
###Code
from sklearn import metrics
from sklearn.neighbors import KNeighborsClassifier
knn = KNeighborsClassifier(n_neighbors=5,metric='minkowski',p=2)
knn.fit(X_train_scaled,y_train)
y_pred_knn = knn.predict(X_test_scaled)
print(confusion_matrix(y_test,y_pred_knn))
print(accuracy_score(y_test,y_pred_knn))
###Output
[[4036 123]
[ 399 120]]
0.8884138520735357
###Markdown
**SVM**
###Code
from sklearn.svm import SVC
svc = SVC(kernel='linear',random_state=0)
svc.fit(X_train_scaled,y_train)
y_pred_svm = svc.predict(X_test)
cmsvc = confusion_matrix(y_test,y_pred_svm)
accuracy = accuracy_score(y_test,y_pred_svm)
print(cmsvc)
print(accuracy)
###Output
[[4159 0]
[ 519 0]]
0.8890551517742625
###Markdown
**Kernal SVM**
###Code
from sklearn.svm import SVC
kernel = SVC(kernel='rbf',random_state=0)
kernel.fit(X_train_scaled,y_train)
y_pred_kernal = kernel.predict(X_test_scaled)
cmkernal = confusion_matrix(y_test,y_pred_kernal)
accuracy = accuracy_score(y_test,y_pred_kernal)
print(cmkernal)
print(accuracy)
###Output
[[4090 69]
[ 416 103]]
0.8963232150491663
###Markdown
**Naive Bayes Model**
###Code
from sklearn.naive_bayes import GaussianNB
bayes = GaussianNB()
bayes.fit(X_train_scaled,y_train)
y_pred_NB = bayes.predict(X_test_scaled)
cmNB = confusion_matrix(y_test,y_pred_NB)
accuracy = accuracy_score(y_test,y_pred_NB)
print(cmNB)
print(accuracy)
###Output
[[3834 325]
[ 296 223]]
0.8672509619495511
###Markdown
**Decision Tree Classification**
###Code
from sklearn.tree import DecisionTreeClassifier
dtc = DecisionTreeClassifier(criterion='entropy',random_state =0)
dtc.fit(X_train_scaled,y_train)
y_pred_DTC = dtc.predict(X_test_scaled)
cmdtc = confusion_matrix(y_test,y_pred_DTC)
accuracy = accuracy_score(y_test,y_pred_DTC)
print(cmdtc)
print(accuracy)
###Output
[[3793 366]
[ 341 178]]
0.8488670371953826
###Markdown
**Random Forest Classification**
###Code
from sklearn.ensemble import RandomForestClassifier
rfc = RandomForestClassifier(n_estimators=100,random_state=0)
rfc.fit(X_train,y_train)
y_pred_rfc = rfc.predict(X_test)
cmrfc = confusion_matrix(y_test,y_pred_rfc)
accuracy = accuracy_score(y_test,y_pred_rfc)
print(cmrfc)
print(accuracy)
###Output
[[4074 85]
[ 408 111]]
0.8946130825138948
###Markdown
Accuracy Scores of All Models >XGB Classification --> 0.89867>Logistic Regression --> 0.89867>KNN Classifier --> 0.88841>SVM Classification --> 0.88905>Kernal SVM --> 0.89632>Naive Bayes --> 0.86725>Decision Tree Classifier --> 0.84886>Random Forest Classification --> 0.89461 - From above result both XGB and Logistic Regression gives same accuracy. Thus, Choose any of these predict the new Customer's List Predicting the `New` Customer List
###Code
newDF = pd.read_excel("New_customer_list_data.xlsx")
newDF.head()
newDF.shape
newDF.isnull().sum()
TdF = newDF.copy()
TdF['customer_age'] = TdF['customer_age'].replace(np.NaN,int(TdF['customer_age'].mean()))
TdF['personal_loan'] = TdF['personal_loan'].replace(np.NaN,'no')
num = TdF['num_contacts_in_campaign'].values.reshape(len(TdF),1)
prev = TdF['num_contacts_prev_campaign'].values.reshape(len(TdF),1)
index = []
for i in np.argwhere(np.isnan(np.concatenate((num,prev),axis=1))):
index.append(i[0])
con = np.concatenate((num,prev),axis=1)
for i in index:
con[i][0] = con[i][1]
dic = {}
for i in range(0,len(index)):
dic[index[i]] = con[i][0]
n = TdF['num_contacts_in_campaign'].values
for i in range(0,len(n)):
if i in dic:
n[i] = dic[i]
TdF['num_contacts_in_campaign'] = pd.Series(n)
TdF.head()
TdF = TdF.dropna()
TdF2 = TdF.copy()
numerical_features = [feature for feature in TdF2.columns if ((TdF2[feature].dtypes != 'O') & (feature not in ['term_deposit_subscribed']))]
print("Number of numerical variable : ",len(numerical_features))
TdF2[numerical_features].head()
discrete_feature = [feature for feature in numerical_features if len(TdF2[feature].unique()) < 25]
print("Descrete variables Count : {}".format(len(discrete_feature)))
continuous_features = [feature for feature in numerical_features if feature not in discrete_feature+['term_deposit_subscribed']]
print("Continuous feature Count {}".format(len(continuous_features)))
TdF2 = TdF2.drop(['default'],axis=1)
TdF3 = TdF2[TdF2['num_contacts_in_campaign'] < 22]
TdF4 = TdF3[TdF3['num_contacts_in_campaign'] < 22]
cat_columns = ['job_type','marital','education','communication_type','month','prev_campaign_outcome']
for col in cat_columns:
TdF4 = pd.concat([TdF4.drop(col,axis=1),pd.get_dummies(TdF4[col],prefix=col,prefix_sep="_",drop_first=True,dummy_na=False)],axis=1)
bool_columns = ['housing_loan', 'personal_loan']
for col in bool_columns:
TdF4[col+'_new']=TdF4[col].apply(lambda x : 1 if x == 'yes' else 0)
TdF4.drop(col, axis=1,inplace=True)
TdF5 = TdF4.copy()
TdF5.iloc[0,:].values
result = model_xgb.predict(TdF5.iloc[:,1:].values)
print(len(result))
###Output
7562
###Markdown
> `1000` customers list who might be intrested for term deposit account
###Code
final = []
count = 0
for item in zip(TdF5.iloc[:,0].values,result):
if item[1] == 1:
final.append(list(item))
count += 1
if count == 1000:
break
final
###Output
_____no_output_____
###Markdown
`1000` customers list who might be intrested for term deposit account
###Code
dFinal = pd.DataFrame(final,columns=['customer_id','status'])
dFinal.to_excel('result.xlsx')
###Output
_____no_output_____ |
deep_learning_v2_pytorch/keras/student-admissions-keras/StudentAdmissionsKeras.ipynb | ###Markdown
Predicting Student Admissions with Neural Networks in KerasIn this notebook, we predict student admissions to graduate school at UCLA based on three pieces of data:- GRE Scores (Test)- GPA Scores (Grades)- Class rank (1-4)The dataset originally came from here: http://www.ats.ucla.edu/ Loading the dataTo load the data and format it nicely, we will use two very useful packages called Pandas and Numpy. You can read on the documentation here:- https://pandas.pydata.org/pandas-docs/stable/- https://docs.scipy.org/
###Code
# Importing pandas and numpy
import pandas as pd
import numpy as np
# Reading the csv file into a pandas DataFrame
data = pd.read_csv("student_data.csv")
# Printing out the first 10 rows of our data
data[:10]
###Output
_____no_output_____
###Markdown
Plotting the dataFirst let's make a plot of our data to see how it looks. In order to have a 2D plot, let's ingore the rank.
###Code
# Importing matplotlib
import matplotlib.pyplot as plt
# Function to help us plot
def plot_points(data):
X = np.array(data[["gre", "gpa"]])
y = np.array(data["admit"])
admitted = X[np.argwhere(y == 1)]
rejected = X[np.argwhere(y == 0)]
plt.scatter(
[s[0][0] for s in rejected],
[s[0][1] for s in rejected],
s=25,
color="red",
edgecolor="k",
)
plt.scatter(
[s[0][0] for s in admitted],
[s[0][1] for s in admitted],
s=25,
color="cyan",
edgecolor="k",
)
plt.xlabel("Test (GRE)")
plt.ylabel("Grades (GPA)")
# Plotting the points
plot_points(data)
plt.show()
###Output
_____no_output_____
###Markdown
Roughly, it looks like the students with high scores in the grades and test passed, while the ones with low scores didn't, but the data is not as nicely separable as we hoped it would. Maybe it would help to take the rank into account? Let's make 4 plots, each one for each rank.
###Code
# Separating the ranks
data_rank1 = data[data["rank"] == 1]
data_rank2 = data[data["rank"] == 2]
data_rank3 = data[data["rank"] == 3]
data_rank4 = data[data["rank"] == 4]
# Plotting the graphs
plot_points(data_rank1)
plt.title("Rank 1")
plt.show()
plot_points(data_rank2)
plt.title("Rank 2")
plt.show()
plot_points(data_rank3)
plt.title("Rank 3")
plt.show()
plot_points(data_rank4)
plt.title("Rank 4")
plt.show()
###Output
_____no_output_____
###Markdown
This looks more promising, as it seems that the lower the rank, the higher the acceptance rate. Let's use the rank as one of our inputs. In order to do this, we should one-hot encode it. One-hot encoding the rankFor this, we'll use the `get_dummies` function in pandas.
###Code
# Make dummy variables for rank
one_hot_data = pd.concat([data, pd.get_dummies(data["rank"], prefix="rank")], axis=1)
# Drop the previous rank column
one_hot_data = one_hot_data.drop("rank", axis=1)
# Print the first 10 rows of our data
one_hot_data[:10]
###Output
_____no_output_____
###Markdown
Scaling the dataThe next step is to scale the data. We notice that the range for grades is 1.0-4.0, whereas the range for test scores is roughly 200-800, which is much larger. This means our data is skewed, and that makes it hard for a neural network to handle. Let's fit our two features into a range of 0-1, by dividing the grades by 4.0, and the test score by 800.
###Code
# Copying our data
processed_data = one_hot_data[:]
# Scaling the columns
processed_data["gre"] = processed_data["gre"] / 800
processed_data["gpa"] = processed_data["gpa"] / 4.0
processed_data[:10]
###Output
_____no_output_____
###Markdown
Splitting the data into Training and Testing In order to test our algorithm, we'll split the data into a Training and a Testing set. The size of the testing set will be 10% of the total data.
###Code
sample = np.random.choice(
processed_data.index, size=int(len(processed_data) * 0.9), replace=False
)
train_data, test_data = processed_data.iloc[sample], processed_data.drop(sample)
print("Number of training samples is", len(train_data))
print("Number of testing samples is", len(test_data))
print(train_data[:10])
print(test_data[:10])
###Output
Number of training samples is 360
Number of testing samples is 40
admit gre gpa rank_1 rank_2 rank_3 rank_4
81 0 0.775 0.7675 0 1 0 0
110 0 0.850 0.7700 0 0 0 1
156 0 0.700 0.6300 0 1 0 0
261 0 0.550 0.7875 0 1 0 0
12 1 0.950 1.0000 1 0 0 0
391 1 0.825 0.9700 0 1 0 0
169 0 0.750 0.9050 0 0 1 0
3 1 0.800 0.7975 0 0 0 1
101 0 0.725 0.8925 0 0 1 0
35 0 0.500 0.7625 0 1 0 0
admit gre gpa rank_1 rank_2 rank_3 rank_4
6 1 0.700 0.7450 1 0 0 0
9 0 0.875 0.9800 0 1 0 0
38 1 0.625 0.7825 0 1 0 0
44 0 0.875 0.7350 0 1 0 0
46 1 0.725 0.8650 0 1 0 0
50 0 0.800 0.9650 0 0 1 0
64 0 0.725 1.0000 0 0 1 0
67 0 0.775 0.8250 1 0 0 0
68 0 0.725 0.9225 1 0 0 0
69 0 1.000 0.9325 1 0 0 0
###Markdown
Splitting the data into features and targets (labels)Now, as a final step before the training, we'll split the data into features (X) and targets (y).Also, in Keras, we need to one-hot encode the output. We'll do this with the `to_categorical function`.
###Code
import keras
# Separate data and one-hot encode the output
# Note: We're also turning the data into numpy arrays, in order to train the model in Keras
features = np.array(train_data.drop("admit", axis=1))
targets = np.array(keras.utils.to_categorical(train_data["admit"], 2))
features_test = np.array(test_data.drop("admit", axis=1))
targets_test = np.array(keras.utils.to_categorical(test_data["admit"], 2))
print(features[:10])
print(targets[:10])
###Output
Using TensorFlow backend.
###Markdown
Defining the model architectureHere's where we use Keras to build our neural network.
###Code
# Imports
import numpy as np
from keras.models import Sequential
from keras.layers.core import Dense, Dropout, Activation
from keras.optimizers import SGD
from keras.utils import np_utils
# Building the model
model = Sequential()
model.add(Dense(128, activation="relu", input_shape=(6,)))
model.add(Dropout(0.2))
model.add(Dense(64, activation="relu"))
model.add(Dropout(0.1))
model.add(Dense(2, activation="softmax"))
# Compiling the model
model.compile(loss="categorical_crossentropy", optimizer="adam", metrics=["accuracy"])
model.summary()
###Output
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
dense_1 (Dense) (None, 128) 896
_________________________________________________________________
dropout_1 (Dropout) (None, 128) 0
_________________________________________________________________
dense_2 (Dense) (None, 64) 8256
_________________________________________________________________
dropout_2 (Dropout) (None, 64) 0
_________________________________________________________________
dense_3 (Dense) (None, 2) 130
=================================================================
Total params: 9,282
Trainable params: 9,282
Non-trainable params: 0
_________________________________________________________________
###Markdown
Training the model
###Code
# Training the model
model.fit(features, targets, epochs=200, batch_size=100, verbose=0)
###Output
_____no_output_____
###Markdown
Scoring the model
###Code
# Evaluating the model on the training and testing set
score = model.evaluate(features, targets)
print("\n Training Accuracy:", score[1])
score = model.evaluate(features_test, targets_test)
print("\n Testing Accuracy:", score[1])
###Output
32/360 [=>............................] - ETA: 0s
Training Accuracy: 0.722222222222
32/40 [=======================>......] - ETA: 0s
Testing Accuracy: 0.575
|
notebooks/metric_v1.ipynb | ###Markdown
Metric v1Create a simple "score" to assign our known binaries, just based on the Color-Mag DiagramMaybe get "fancy" and include orbital period
###Code
%matplotlib inline
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
from matplotlib.colors import LogNorm
import matplotlib
matplotlib.rcParams.update({'font.size':18})
# matplotlib.rcParams.update({'font.family':'serif'})
# for the TESS Science Online 48hr sprint, we'll be using Cyberpunk for the graphics!
# https://github.com/dhaitz/mplcyberpunk
import mplcyberpunk
plt.style.use("cyberpunk")
# smooth both EBs and Stars w/ a 2D Gaussian KDE
from sklearn.neighbors import KernelDensity
def kde2D(x, y, bandwidth, xmin=-1, xmax = 5.5, ymin= -6, ymax=16,
xbins=100j, ybins=100j, **kwargs):
"""Build 2D kernel density estimate (KDE)
https://stackoverflow.com/a/41639690"""
# create grid of sample locations (default: 100x100)
xx, yy = np.mgrid[xmin:xmax:xbins,
ymin:ymax:ybins]
xy_sample = np.vstack([yy.ravel(), xx.ravel()]).T
xy_train = np.vstack([y, x]).T
kde_skl = KernelDensity(bandwidth=bandwidth, **kwargs)
kde_skl.fit(xy_train)
# score_samples() returns the log-likelihood of the samples
z = np.exp(kde_skl.score_samples(xy_sample))
return xx, yy, np.reshape(z, xx.shape)
numerator = pd.read_csv('BigCat.csv')
denominator = pd.read_csv('gaia_tess2min.csv')
# EHow = pd.read_csv('EHow.csv')
EHow = pd.read_csv('Erin_and_Known_EBs.csv')
Eok = ((EHow['parallax'] > 0) &
np.isfinite(EHow['bp_rp']) &
np.isfinite(EHow['phot_g_mean_mag']))
plt.figure(figsize=(7,8))
plt.hist2d(EHow['bp_rp'][Eok],
EHow['phot_g_mean_mag'][Eok] - 5. * np.log10(1000./EHow['parallax'][Eok]) + 5,
norm=LogNorm(), cmap=plt.cm.cool, bins=100)
plt.gca().invert_yaxis()
plt.xlabel('$G_{BP} - G_{RP}$ (mag)')
plt.ylabel('$M_G$ (mag)')
plt.grid(True)
# # lets plot the EBs in CMD space
# Nok = ((numerator['parallax'] > 0) &
# np.isfinite(numerator['bp_rp']) &
# np.isfinite(numerator['phot_g_mean_mag']))
# plt.figure(figsize=(7,8))
# plt.hist2d(numerator['bp_rp'][Nok],
# numerator['phot_g_mean_mag'][Nok] - 5. * np.log10(1000./numerator['parallax'][Nok]) + 5,
# norm=LogNorm(), cmap=plt.cm.cool, bins=100)
# plt.gca().invert_yaxis()
# plt.xlabel('$G_{BP} - G_{RP}$ (mag)')
# plt.ylabel('$M_G$ (mag)')
# plt.grid(True)
# mplcyberpunk.add_glow_effects()
# ==> i'm concerned about ASAS-SN... it's over-populated w/ stuff in a big clump!
# adjust the cut to drop ASAS-SN for now
Nok = ((numerator['parallax'] > 0) &
(1000./numerator['parallax']< 40000) &
np.isfinite(numerator['bp_rp']) &
np.isfinite(numerator['phot_g_mean_mag']) &
(np.arange(len(numerator)) < 12520)
) # all the EBs except ASAS-SN
print(sum(Nok))
plt.figure(figsize=(7,8))
plt.hist2d(numerator['bp_rp'][Nok],
numerator['phot_g_mean_mag'][Nok] - 5. * np.log10(1000./numerator['parallax'][Nok]) + 5,
norm=LogNorm(), cmap=plt.cm.cool, bins=100)
plt.gca().invert_yaxis()
plt.xlabel('$G_{BP} - G_{RP}$ (mag)')
plt.ylabel('$M_G$ (mag)')
plt.grid(True)
mplcyberpunk.add_glow_effects()
# lets plot all the TESS stars in CMD space
Dok = ((denominator['parallax'] > 0) &
np.isfinite(denominator['bp_rp']) &
np.isfinite(denominator['phot_g_mean_mag']))
plt.figure(figsize=(7,8))
plt.hist2d(denominator['bp_rp'][Dok],
denominator['phot_g_mean_mag'][Dok] - 5. * np.log10(1000./denominator['parallax'][Dok]) + 5,
norm=LogNorm(), cmap=plt.cm.cool, bins=100)
plt.gca().invert_yaxis()
plt.xlabel('$G_{BP} - G_{RP}$ (mag)')
plt.ylabel('$M_G$ (mag)')
plt.grid(True)
mplcyberpunk.add_glow_effects()
# xx1, yy1, zz1 = kde2D(numerator['bp_rp'][Nok],
# numerator['phot_g_mean_mag'][Nok] - 5. * np.log10(1000./numerator['parallax'][Nok]) + 5,
# 0.2)
xx1, yy1, zz1 = kde2D(EHow['bp_rp'][Eok],
EHow['phot_g_mean_mag'][Eok] - 5. * np.log10(1000./EHow['parallax'][Eok]) + 5,
0.1)
plt.figure(figsize=(7,8))
plt.pcolormesh(xx1, yy1, zz1, cmap=plt.cm.Spectral_r)
plt.gca().invert_yaxis()
plt.xlabel('$G_{BP} - G_{RP}$ (mag)')
plt.ylabel('$M_G$ (mag)')
plt.grid(True)
plt.title('TESS 2-min EBs')
xx2, yy2, zz2 = kde2D(denominator['bp_rp'][Dok],
denominator['phot_g_mean_mag'][Dok] - 5. * np.log10(1000./denominator['parallax'][Dok]) + 5,
0.1)
plt.pcolormesh(xx2, yy2, zz2, cmap=plt.cm.Spectral_r)
plt.gca().invert_yaxis()
plt.title('TESS Background')
plt.xlabel('$G_{BP} - G_{RP}$ (mag)')
plt.ylabel('$M_G$ (mag)')
plt.grid(True)
plt.figure(figsize=(7,8))
plt.pcolormesh(xx2, yy2, zz1, cmap=plt.cm.Spectral_r)
plt.gca().invert_yaxis()
plt.xlabel('$G_{BP} - G_{RP}$ (mag)')
plt.ylabel('$M_G$ (mag)')
plt.grid(True)
plt.contour(xx2, yy2, zz2/np.sum(zz2)*np.float(len(Dok)),
colors='white', levels=(1,3,10,30,70,100,200), alpha=0.5)
SCORE = (1-(zz1+1)/(zz2+1))*100
# SCORE = (np.log10(zz1/np.sum(zz1)*np.float(len(Dok))+1e-9) -
# np.log10(zz2/np.sum(zz2)*np.float(len(Dok))+1e-9) )
# center about SCORE=0...
if -np.min(SCORE) > np.max(SCORE):
SCORE[-SCORE > np.max(SCORE)] = -np.max(SCORE)
if np.max(SCORE) > -np.min(SCORE):
SCORE[np.max(SCORE) > -np.min(SCORE)] = -np.min(SCORE)
plt.figure(figsize=(7,8))
plt.pcolormesh(xx2, yy2, SCORE, cmap=plt.cm.cool)
plt.gca().invert_yaxis()
plt.xlabel('$G_{BP} - G_{RP}$ (mag)')
plt.ylabel('$M_G$ (mag)')
plt.grid(True)
cb=plt.colorbar()
cb.set_label('Score')
# plt.contour(xx2, yy2, (zz1+1)/(zz2+1), colors='white')
plt.contour(xx2, yy2, zz2/np.sum(zz2)*np.float(len(Dok)),
colors='white', levels=(1,3,10,30,70,100,200), alpha=0.5)
x = EHow['bp_rp'][Eok]
y = EHow['phot_g_mean_mag'][Eok] - 5. * np.log10(1000./EHow['parallax'][Eok]) + 5
plt.xlim(min(x), max(x))
plt.ylim(max(y), min(y))
plt.savefig('score_v1.png', dpi=300, bbox_inches='tight', pad_inches=0.25)
###Output
/Users/james/opt/anaconda3/lib/python3.7/site-packages/ipykernel_launcher.py:14: MatplotlibDeprecationWarning: shading='flat' when X and Y have the same dimensions as C is deprecated since 3.3. Either specify the corners of the quadrilaterals with X and Y, or pass shading='auto', 'nearest' or 'gouraud', or set rcParams['pcolor.shading']. This will become an error two minor releases later.
###Markdown
here are the boundary conditions i *want* to satisfy: Score == 1 if there are no EBs, regardless of of background stars!Score == 0 if there are lots of EBs relative to the of background stars
###Code
# _ = plt.hist(1000./numerator['parallax'][Nok], density=True, alpha=0.7, bins=np.linspace(0,4000,25), label='EBs')
# _ = plt.hist(1000./denominator['parallax'][Dok], density=True, alpha=0.7, bins=np.linspace(0,4000,25), label='TESS stars')
# _ = plt.hist(1000./EHow['parallax'][Eok], density=True, alpha=0.7, bins=np.linspace(0,4000,25), label='E Howard EBs')
# plt.xlabel('Distance [pc]')
# plt.legend(fontsize=13)
# OK, i think this bimodal blue/red score we're getting at bp-rp=1 is b/c the samples are
# drawn from radically different [Fe/H] distributions, and separating in the CMD
# X = np.vstack((EHow['bp_rp'][Eok].values,
# EHow['phot_g_mean_mag'][Eok].values - 5. * np.log10(1000./EHow['parallax'][Eok].values) + 5)).T
# X.shape
# k = 50
# from sklearn.cluster import KMeans
# kmeans = KMeans(n_clusters=k, init='random', n_init=1, random_state=0, max_iter=1)
# kmeans.fit(X)
# y_kmeans = kmeans.predict(X) # cluster index for each observation
# centers = kmeans.cluster_centers_ # cluster center coordinates
# plt.scatter(X[:, 0], X[:, 1], c=y_kmeans, s=5, cmap='summer')
# plt.scatter(centers[:, 0], centers[:, 1], c='black', s=100, alpha=0.5)
# plt.gca().invert_yaxis()
# from scipy.spatial import Voronoi, voronoi_plot_2d
# vor = Voronoi(centers)
# voronoi_plot_2d(vor)
# plt.scatter(X[:, 0], X[:, 1], c=y_kmeans, s=5, cmap='summer')
# plt.gca().invert_yaxis()
# from voronoi.voronoi import bin2d
# xp = EHow['bp_rp'][Eok].values
# yp = EHow['phot_g_mean_mag'][Eok].values - 5. * np.log10(1000./EHow['parallax'][Eok].values) + 5
# pix_bin, bin_x, bin_y, bin_sn, bin_npix, scale = \
# bin2d(xp, yp, np.ones_like(xp), np.zeros_like(xp)+.1, 10.,
# cvt=False, wvt=False, graphs=False, quiet=True)
# # Bin stats
# bad = bin_sn < 5
# masked = pix_bin*1
# mean_bins = pix_bin*0.
# median_bins = pix_bin*0.
# mea = bin_x*0.
# med = bin_x*0.
# bx = bin_x*0.
# by = bin_y*0.
# bin_ids = np.unique(pix_bin)
# for i in range(len(bin_ids)):
# bin_mask = pix_bin == bin_ids[i]
# mea[i] = np.ones_like(xp)[bin_mask].sum()
# mean_bins[bin_mask] = mea[i]
# med[i] = np.sum(np.ones_like(xp)[bin_mask])
# median_bins[bin_mask] = med[i]
# bx[i] = np.sum(xp*bin_mask)/bin_mask.sum()
# by[i] = np.sum(yp*bin_mask)/bin_mask.sum()
# for bin in np.where(bad)[0]:
# bin_mask = pix_bin == bin
# masked[bin_mask] = -99
# # Plot
# plt.rcParams['image.origin'] = 'lower'
# # fig = plt.figure(figsize=[9, 2.8])
# # ax = fig.add_subplot(131)
# # ax.imshow(pix_bin.reshape(xp.shape))
# # ax.scatter(bin_x, bin_y, marker='.', color='k', alpha=0.1)
# plt.scatter(bin_x, bin_y)
# # ax = fig.add_subplot(132)
# # ax.imshow(np.ones_like(xp), vmin=-0.1, vmax=10, cmap='gray_r')
# # ax = fig.add_subplot(133)
# # ax.imshow(median_bins.reshape(xp.shape), vmin=-0.1, vmax=10, cmap='gray_r')
# # try Voronoi pixels to bin up our sample?
# # https://pypi.org/project/vorbin/
# from vorbin.voronoi_2d_binning import voronoi_2d_binning
# binNum, xBin, yBin, xBar, yBar, sn, nPixels, scale = \
# voronoi_2d_binning(EHow['bp_rp'][Eok].values,
# EHow['phot_g_mean_mag'][Eok].values - 5. * np.log10(1000./EHow['parallax'][Eok].values) + 5,
# np.ones_like(EHow['bp_rp'][Eok].values),
# np.zeros_like(EHow['bp_rp'][Eok].values)+.1,
# 10)
###Output
_____no_output_____ |
2019/Day 19.ipynb | ###Markdown
Day 19 - Tractor beam scanning* https://adventofcode.com/2019/day/19This starts as a simple intcode excercise; count the number of 1's in a 50x50 grid. The CPU programme halts after each coordinate, so we need to run the programme in a loop.
###Code
from __future__ import annotations
from itertools import product
from typing import (
List,
)
import numpy as np
from IPython.display import display, DisplayHandle
from PIL import Image, ImageDraw
from intcode import CPU, ioset
def measure_signal(memory: List[int], scale=5, size=50, x: int = 0, y: int = 0) -> np.array:
image = Image.new('1', (size * scale, size * scale))
draw = ImageDraw.Draw(image)
dh = display(image, display_id=True)
matrix = np.zeros((size, size), dtype=np.bool)
for yd, xd in product(range(size), repeat=2):
xx, yy = x + xd, y + yd
outputs, opcodes = ioset(xx, yy)
CPU(opcodes).reset(memory).execute()
value = outputs[0]
draw.rectangle((xd * scale, yd * scale, (xd + 1) * scale, (yd + 1) * scale), value)
dh.update(image)
matrix[yd, xd] = bool(value)
return matrix
import aocd
data = aocd.get_data(day=19, year=2019)
memory = list(map(int, data.split(',')))
matrix = measure_signal(memory)
print("Part 1:", matrix.sum())
###Output
_____no_output_____
###Markdown
Part 2 - Find where the beam is wide enoughScanning a 50x50 area took long enough; finding that *really large area* is going to taka a lot longer. Since the beam is widenining at a steady rate, we should be able to calculate where we'll be able to fit a 100x100 grid in the beam, however.If the beam indeed widens at a constant rate, then the lines are simply two functions over y; both lines start at 0, so we only need to know the slopes $a$ and $b$:$$y_t(x) = ax \\y_b(x) = bx$$The two slopes start at the same point, $(0, 0)$ and diverge. When the distance between this is $99 + (99 * a)$, then we can fit a 100 x 100 square in between, because that's the point fromhich the top line (with slope $a$) still has enough space to descend and accomodate the top right-hand corner.So we can find the left-hand $x$ where $bx = ax + 99 + (99 * a)$, which can be simplified to:$$x = \frac{99(a + 1)}{a - b}$$We'll first need to determine the slopes $a$ and $b$ from the information the drone can give us. We can detect the transition from 0 to 1 and from 1 to zero at two separate $x$ coordinates for that, to give us the corresponding $y$ coordinates. Using numpy it's a simple subtraction of the shifted matrix; -1 markes the point from 0 -> 1 and 1 markes it from 1 -> 0. Then find the indices of the minimums (the -1 values), and the indices of the maximums. The latter are the point where it goes back into black, so the actual line needs 1 subtracted.I've added a 'calibration' function that checks the values with the drone until we can predict the values exactly.
###Code
from fractions import Fraction
from itertools import count, groupby
from operator import itemgetter
def measure_x(memory: List[int], x: int, y_range: range) -> Iterator[int]:
for y in y_range:
outputs, opcodes = ioset(x, y)
CPU(opcodes).reset(memory).execute()
yield outputs[0]
def calibrate(memory: List[int], x: int, y: int, top: bool = True, tolerance: int = 5) -> int:
yr = range(y - tolerance, y + tolerance + 1)
yc = next(next(yvs)[0] for v, yvs in groupby(
enumerate(measure_x(memory, x, yr), yr.start),
itemgetter(1)
) if v == int(top))
return yc
def find_slopes(matrix: np.array, memory: List[int]) -> Tuple[Fraction, Fraction]:
"""Find the rate at which y declines over x, for the top and bottom lines"""
matrix = matrix.view(np.int8) # signed type to make sure we can get -1
delta_yt = (matrix[1:, :] - matrix[:-1, :]).argmax(axis=0) + 1
delta_yb = (matrix[1:, :] - matrix[:-1, :]).argmin(axis=0) + 1
a, b = Fraction(delta_yt[-1], 49), Fraction(delta_yb[-1], 49)
# calibrate by reading out larger values until a and b are stable
for x1 in count(1000, 1000):
yt1 = x1 * a.numerator // a.denominator
ytc = calibrate(memory, x1, yt1)
yb1 = (x1 * b.numerator // b.denominator) + 1
ybc = calibrate(memory, x1, yb1, False)
if yt1 == ytc and yb1 == ybc:
return a, b
a = Fraction(ytc, x1)
b = Fraction(ybc, x1)
def find_fit(matrix: np.array, memory: List[int]) -> int:
a, b = find_slopes(matrix, memory)
xb = round((99 * (a + 1)) / (b - a))
yb = xb * b.numerator // b.denominator
xt = xb + 99
yt = xt * a.numerator // a.denominator
return xb * 10000 + yt
print("Part 2:", find_fit(matrix, memory))
###Output
Part 2: 9760485
|
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.