id
int64 393k
2.82B
| repo
stringclasses 68
values | title
stringlengths 1
936
| body
stringlengths 0
256k
⌀ | labels
stringlengths 2
508
| priority
stringclasses 3
values | severity
stringclasses 3
values |
---|---|---|---|---|---|---|
278,666,346 | go | cmd/compile: eliminate runtime.conv* calls with unused results | ### What version of Go are you using (`go version`)?
`go version go1.9.2 linux/amd64`
### Does this issue reproduce with the latest release?
Yes
### What operating system and processor architecture are you using (`go env`)?
`linux/amd64`
### What did you do?
I'm using the following file (`noop.go`):
```go
package main
import "fmt"
func debug(data ...interface{}) {}
func op() {
fmt.Println("Op")
}
func main() {
debug("123")
op()
}
```
I compile the file using: `go tool compile noop.go`.
Then I inspect the object file using: `go tool objdump noop.o`.
### What did you expect to see?
Ideally I expect to see no traces of the `debug` function.
In a non-ideal, but a good outcome I expect to see no calls to the `debug` function.
### What did you see instead?
A call to the `debug` function.
```
noop.go:12 0x569 e800000000 CALL 0x56e [1:5]R_CALL:%22%22.debug
noop.go:13 0x56e e800000000 CALL 0x573 [1:5]R_CALL:%22%22.op
```
### Additional information
If I replace signature of the `debug` function to:
```go
func debug(data string) {}
```
I get the "good" scenarion:
* the `debug` function is present
* no calls to the `debug` function exist | Performance,NeedsFix,binary-size | medium | Critical |
278,718,030 | rust | DWARF: linkage_name does not include hash, does not match any symbol | So this is going to be a long issue, but the gist is, to put it semi dramatically, is that I think that all of rust debugging info might be slightly broken, but workable enough for say gdb, that it has gone unnoticed. At the very least, I think:
1. no_mangle statics are definitely broken https://github.com/rust-lang/rust/issues/33172
2. there are some major discrepancies between rust and c++ dwarf output that should be resolved, w.r.t. the DWARF `linkage_name`
/cc @philipc @fitzgen @tromey @rkruppe @michaelwoerister
# Discussion
I've created some test files in rust and c++, and also grepped the binaries for dwarf dies and also symbol table values, which I explain below.
## Repro / Test files
```rust
#[derive(Debug)]
pub struct Foo {
x: u64,
y: i32,
}
#[no_mangle]
pub static TEST: Foo = Foo { x: 0xdeadbeef, y: -55 };
pub static TEST2: Foo = Foo { x: 0xbeefdead, y: -55 };
fn deadbeef() {
println!("TEST: {:?} - {:?}", TEST, TEST2);
}
pub fn main() {
deadbeef()
}
```
and an approximating C++ file:
```c++
#include<cstdio>
#include<cstdint>
namespace test {
struct Foo {
uint64_t x;
int64_t y;
};
Foo TEST = { .x = 0xdeadbeef, .y = -55 };
}
test::Foo TEST = { .x = 0xbeefdead, .y = -55 };
namespace test {
void deadbeef() {
printf("test::TEST: {0x%lx, %ld}\n", test::TEST.x, test::TEST.y);
printf("TEST: {0x%lx, %ld}\n", TEST.x, TEST.y);
}
}
int main() {
test::deadbeef();
return 0;
}
```
I've compiled the rust and c++ versions as follows:
```
rustc -g test.rs -o test
g++ -g -std=c++11 test.cpp -o test_cpp
clang++ -g -std=c++11 test.cpp -o test_cpp_clang
```
I will use the clang output for the c++ examples below, since it shares the same backend infrastructure, though the g++ does output the same.
## Analysis
First I will show the dwarf values for `TEST`, `TEST2`, and the function `deadbeef` for the `test` binary, then the `test_cpp_clang` binary.
I would like to direct the reader's attention to the `DW_AT_linkage_name` field, and the corresponding linkage name it shows for each binary.
```
DW_TAG_variable [3]
DW_AT_name [DW_FORM_strp] ( .debug_str[0x00000061] = "TEST")
DW_AT_type [DW_FORM_ref4] (cu + 0x0049 => {0x00000049})
DW_AT_external [DW_FORM_flag_present] (true)
DW_AT_decl_file [DW_FORM_data1] ("/home/m4b/tmp/bad_debug/test.rs")
DW_AT_decl_line [DW_FORM_data1] (8)
DW_AT_alignment [DW_FORM_udata] (1)
DW_AT_location [DW_FORM_exprloc] (<0x9> 03 c0 65 05 00 00 00 00 00 )
DW_AT_linkage_name [DW_FORM_strp] ( .debug_str[0x00000066] = "_ZN4test4TESTE")
DW_TAG_variable [6]
DW_AT_name [DW_FORM_strp] ( .debug_str[0x00000075] = "TEST2")
DW_AT_type [DW_FORM_ref4] (cu + 0x0049 => {0x00000049})
DW_AT_decl_file [DW_FORM_data1] ("/home/m4b/tmp/bad_debug/test.rs")
DW_AT_decl_line [DW_FORM_data1] (10)
DW_AT_alignment [DW_FORM_udata] (1)
DW_AT_location [DW_FORM_exprloc] (<0x9> 03 d0 65 05 00 00 00 00 00 )
DW_AT_linkage_name [DW_FORM_strp] ( .debug_str[0x0000007b] = "_ZN4test5TEST2E")
DW_TAG_subprogram [7] *
DW_AT_low_pc [DW_FORM_addr] (0x00000000000073b0)
DW_AT_high_pc [DW_FORM_data4] (0x000000f7)
DW_AT_frame_base [DW_FORM_exprloc] (<0x1> 56 )
DW_AT_linkage_name [DW_FORM_strp] ( .debug_str[0x000000d9] = "_ZN4test8deadbeefE")
DW_AT_name [DW_FORM_strp] ( .debug_str[0x000000ec] = "deadbeef")
DW_AT_decl_file [DW_FORM_data1] ("/home/m4b/tmp/bad_debug/test.rs")
DW_AT_decl_line [DW_FORM_data1] (12)
```
And now for the cpp version:
```
DW_TAG_variable [3]
DW_AT_name [DW_FORM_strp] ( .debug_str[0x00000053] = "TEST")
DW_AT_type [DW_FORM_ref4] (cu + 0x0048 => {0x00000048})
DW_AT_external [DW_FORM_flag_present] (true)
DW_AT_decl_file [DW_FORM_data1] ("/home/m4b/tmp/bad_debug/test.cpp")
DW_AT_decl_line [DW_FORM_data1] (11)
DW_AT_location [DW_FORM_exprloc] (<0x9> 03 30 10 20 00 00 00 00 00 )
DW_AT_linkage_name [DW_FORM_strp] ( .debug_str[0x0000008e] = "_ZN4test4TESTE")
DW_TAG_subprogram [6]
DW_AT_low_pc [DW_FORM_addr] (0x0000000000000670)
DW_AT_high_pc [DW_FORM_data4] (0x0000004c)
DW_AT_frame_base [DW_FORM_exprloc] (<0x1> 56 )
DW_AT_linkage_name [DW_FORM_strp] ( .debug_str[0x000002be] = "_ZN4test8deadbeefEv")
DW_AT_name [DW_FORM_strp] ( .debug_str[0x000002d2] = "deadbeef")
DW_AT_decl_file [DW_FORM_data1] ("/home/m4b/tmp/bad_debug/test.cpp")
DW_AT_decl_line [DW_FORM_data1] (17)
DW_AT_external [DW_FORM_flag_present] (true)
DW_TAG_variable [9]
DW_AT_name [DW_FORM_strp] ( .debug_str[0x00000053] = "TEST")
DW_AT_type [DW_FORM_ref4] (cu + 0x0048 => {0x00000048})
DW_AT_external [DW_FORM_flag_present] (true)
DW_AT_decl_file [DW_FORM_data1] ("/home/m4b/tmp/bad_debug/test.cpp")
DW_AT_decl_line [DW_FORM_data1] (14)
DW_AT_location [DW_FORM_exprloc] (<0x9> 03 40 10 20 00 00 00 00 00 )
```
The first thing to note is that for the rust, `no_mangle` static, `TEST`, it is given a linkage name:
```
DW_AT_linkage_name [DW_FORM_strp] ( .debug_str[0x00000066] = "_ZN4test4TESTE")
```
In contrast to the cpp version, which (correctly) has none. I believe the rust DIE that is emitted is outright incorrect, and is the cause of the issue in https://github.com/rust-lang/rust/issues/33172
Note, although this issue was closed in favor of https://github.com/rust-lang/rust/issues/32574 that issue _does not_ `no_mangle` the static.
Unfortunately, that issue also noted (but did not seem to pursue further):
> Now, this variable is not actually emitted. There's no ELF symbol for it.
which I believe may be the crux of the major problem at large here: the `linkage_name` on **all non-mangled Rust DWARF DIEs** references a **non-existent** symbol - I think this is at best highly unusual, and at worst problematic.
## Missing Symbols
Considering only ELF at the moment, we can verify that for `TEST`, `TEST2`, and `deadbeef`, there _is no symbol_ referenced by the `linkage_name` on the DIE:
```
565d0 LOCAL OBJECT _ZN4test5TEST217h8314c5b1b9028ef4E 0x10 .rodata(16)
73b0 LOCAL FUNC _ZN4test8deadbeef17hc8e13bcb4738fc41E 0xf7 .text(14)
565c0 GLOBAL OBJECT TEST 0x10 .rodata(16)
```
You will note that the symbols include the [symbol hash](https://github.com//m4b/rust/blob/b15a8eafcd7e50af116d398c1ac3c5a0504c0270/src/librustc_trans/back/symbol_names.rs#L152).
In contrast with the cpp version, the symbol name (including parameter types, see the `v` (for void) in `_ZN4test8deadbeefEv`) is identical to the linkage_name, as I think, expected:
```
201040 GLOBAL OBJECT TEST 0x10 .data(22)
670 GLOBAL FUNC _ZN4test8deadbeefEv 0x4c .text(12)
201030 GLOBAL OBJECT _ZN4test4TESTE 0x10 .data(22)
```
# Debuggers
I would now like to present a debugging session (primarily in `gdb`) for the two binaries to attempt to illustrate some of the oddities that occur, and motivate why I think there is something wrong here, at the very least.
There are general ergonomic issues and other oddities that I think are surfacing because of the current debug info situation. I have used `gdb` to illustrate, as `lldb` is essentially non-functioning for me, and when it doesn't segfault, I cannot break on un-mangled names for the rust binaries.
## GDB
First the rust binary:
```
(gdb) ptype TEST
No symbol 'TEST' in current context
(gdb) ptype test::TEST
No symbol 'test::TEST' in current context
(gdb) ptype test::TEST2
Reading in symbols for test.rs...done.
type = struct test::Foo {
x: u64,
y: i32,
}
(gdb) ptype test::deadbeef
type = fn ()
(gdb) whatis TEST
No symbol 'TEST' in current context
(gdb) whatis test::TEST
No symbol 'test::TEST' in current context
(gdb) whatis test::TEST2
type = test::Foo
(gdb) whatis test::deadbeef
type = fn ()
(gdb) info addr TEST
Symbol "TEST" is at 0x565c0 in a file compiled without debugging.
(gdb) info addr test::TEST
No symbol "test::TEST" in current context.
(gdb) info addr test::TEST2
Symbol "test::TEST2" is static storage at address 0x565d0.
(gdb) info addr test::deadbeef
Symbol "test::deadbeef" is a function at address 0x73b0.
(gdb) info addr _ZN4test5TEST217h8314c5b1b9028ef4E
Symbol "test::TEST2" is at 0x565d0 in a file compiled without debugging.
(gdb) info addr _ZN4test8deadbeef17hc8e13bcb4738fc41E
Symbol "test::deadbeef" is at 0x73b0 in a file compiled without debugging.
```
The last two are particularly troubling. This certainly looks like a direct consequence of mapping the unmangled, symbol name + hash, in the ELF symbol table to the mangled-without-hash _linkage_ name in the DWARF DIE.
If we compare this to the exact same c++ debugging sequence, it is exactly what one would expect:
```
(gdb) ptype TEST
type = struct test::Foo {
uint64_t x;
int64_t y;
}
(gdb) ptype test::TEST
type = struct test::Foo {
uint64_t x;
int64_t y;
}
(gdb) ptype test::deadbeef
type = void (void)
(gdb) whatis TEST
type = test::Foo
(gdb) whatis test::TEST
type = test::Foo
(gdb) whatis test::deadbeef
type = void (void)
(gdb) info addr TEST
Symbol "TEST" is static storage at address 0x201040.
(gdb) info addr test::TEST
Symbol "test::TEST" is static storage at address 0x201030.
(gdb) info addr test::deadbeef
Symbol "test::deadbeef()" is a function at address 0x670.
(gdb) info addr _ZN4test4TESTE
Symbol "test::TEST" is static storage at address 0x201030.
(gdb) info addr _ZN4test8deadbeefEv
Symbol "test::deadbeef()" is a function at address 0x670.
```
## LLDB
As of lldb with llvm 5.0.0 I cannot even auto-complete or break on non-mangled names, which further increases my suspicion something is wrong, and the fact that it segfaults occasionally when I auto-complete using `test::` as a seed and the stack trace indicates its in the dwarf DIE parsing logic is equally suspicious:
```
Process 11803 (lldb) of user 1000 dumped core.
Stack trace of thread 11841:
#0 0x00007f4d2e9a4295 _ZN16DWARFCompileUnit6GetDIEEj (liblldb.so.3.8.0)
#1 0x00007f4d2e9a9f60 _ZN14DWARFDebugInfo6GetDIEERK6DIERef (liblldb.so.3.8.0)
#2 0x00007f4d2e9a4265 _ZN16DWARFCompileUnit6GetDIEEj (liblldb.so.3.8.0)
#3 0x00007f4d2e9ac6b0 _ZNK19DWARFDebugInfoEntry13GetAttributesEPK16DWARFCompileUnitN14DWARFFormValue14
#4 0x00007f4d2e9a4f89 _ZN16DWARFCompileUnit12IndexPrivateEPS_N4lldb12LanguageTypeERKN14DWARFFormValue1
#5 0x00007f4d2e9a454c _ZN16DWARFCompileUnit5IndexER9NameToDIES1_S1_S1_S1_S1_S1_S1_ (liblldb.so.3.8.0)
#6 0x00007f4d2e9cab60 _ZNSt17_Function_handlerIFSt10unique_ptrINSt13__future_base12_Result_baseENS2_8_
#7 0x00007f4d2e9caa1a _ZNSt13__future_base13_State_baseV29_M_do_setEPSt8functionIFSt10unique_ptrINS_12
#8 0x00007f4d31feedbf __pthread_once_slow (libpthread.so.0)
#9 0x00007f4d2e9ca5dc _ZNSt13__future_base11_Task_stateISt5_BindIFZN10TaskRunnerIjE7AddTaskIRZN15Symbo
#10 0x00007f4d2ec2fd15 _ZN12_GLOBAL__N_112TaskPoolImpl6WorkerEPS0_ (liblldb.so.3.8.0)
#11 0x00007f4d2c970a6f execute_native_thread_routine (libstdc++.so.6)
#12 0x00007f4d31fe708a start_thread (libpthread.so.0)
#13 0x00007f4d2c3de47f __clone (libc.so.6)
```
# Solutions
I believe 1. is the first that should be attempted, but I am not an expert in the compiler internals.
1. Have the [dwarf name mangler](https://github.com//m4b/rust/blob/b15a8eafcd7e50af116d398c1ac3c5a0504c0270/src/librustc_trans/debuginfo/namespace.rs#L25) include the [symbol hash](https://github.com//m4b/rust/blob/b15a8eafcd7e50af116d398c1ac3c5a0504c0270/src/librustc_trans/back/symbol_names.rs#L152), specifically I think it needs to be appended right about here: https://github.com//m4b/rust/blob/b15a8eafcd7e50af116d398c1ac3c5a0504c0270/src/librustc_trans/debuginfo/namespace.rs#L47
2. Output non-hash versions of symbols into the symbol table that point to the same address, etc., but which reference the non-hash name in the string table. This is a hack imho, and I've already tried this via binary editing and it did not seem to have any noticeable effect.
Eventually, I think that the final, correct solution imho is to exactly mirror the dwarf output of both the gcc and clang backends, which means:
1. Making the linkage_name = the symbol table name
2. Adding parameters, etc., into the symbol table name?
# Final Considerations
I believe I've also found some weirdness w.r.t. other symbol names, specifically:
1. locally scoped statics,
2. impls of traits with certain annotations
examples of which are:
```
_ZN3std10sys_common9backtrace11log_enabled7ENABLED17hc187c5b3618ccb2eE.0.0
_ZN61_$LT$alloc..heap..Heap$u20$as$u20$alloc..allocator..Alloc$GT$3oom17h28fb525969c57bd8E
```
at lines:
1. https://github.com//m4b/rust/blob/b15a8eafcd7e50af116d398c1ac3c5a0504c0270/src/libstd/sys_common/backtrace.rs#L148 (`0.0` appended to a mangled name is not a valid mangled name afaik)
2. https://github.com//m4b/rust/blob/b15a8eafcd7e50af116d398c1ac3c5a0504c0270/src/liballoc/heap.rs#L94-L100 (the symbol name appears to differ widly from the debug name)
, respectively.
But I think this is another story for another time ;)
| A-linkage,A-debuginfo,T-compiler,C-bug | low | Critical |
278,732,877 | node | Use %TypedArray%.prototype.subarray for Buffer.prototype.slice | * **Version**: master
* **Platform**: all
* **Subsystem**: buffer
Currently, [our implementation](https://github.com/nodejs/node/blob/9fb390a1c66c91796626fe199176619ce4a54f62/lib/buffer.js#L992-L1014) of [`Buffer.prototype.slice()`](https://nodejs.org/api/buffer.html#buffer_buf_slice_start_end) operates identically to [`TypedArray.prototype.subarray()`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/TypedArray/subarray) ([spec](https://tc39.github.io/ecma262/#sec-%typedarray%.prototype.subarray)) down to the last minutia (with the assumption that `this` is indeed a `Buffer`, and `Buffer[Symbol.species]` has not been tampered with). Ideally, we should just set `Buffer.prototype.slice` to `Uint8Array.prototype.subarray` instead of reimplementing it. The only problem is performance: `subarray()` is much slower than `slice()`:
<details><summary>diff</summary>
```diff
diff --git a/lib/buffer.js b/lib/buffer.js
index b56c032f9e..112dc22d69 100644
--- a/lib/buffer.js
+++ b/lib/buffer.js
@@ -989,29 +989,7 @@ Buffer.prototype.toJSON = function toJSON() {
};
-function adjustOffset(offset, length) {
- // Use Math.trunc() to convert offset to an integer value that can be larger
- // than an Int32. Hence, don't use offset | 0 or similar techniques.
- offset = Math.trunc(offset);
- // `x !== x`-style conditionals are a faster form of `isNaN(x)`
- if (offset === 0 || offset !== offset) {
- return 0;
- } else if (offset < 0) {
- offset += length;
- return offset > 0 ? offset : 0;
- } else {
- return offset < length ? offset : length;
- }
-}
-
-
-Buffer.prototype.slice = function slice(start, end) {
- const srcLength = this.length;
- start = adjustOffset(start, srcLength);
- end = end !== undefined ? adjustOffset(end, srcLength) : srcLength;
- const newLength = end > start ? end - start : 0;
- return new FastBuffer(this.buffer, this.byteOffset + start, newLength);
-};
+Buffer.prototype.slice = Uint8Array.prototype.subarray;
function checkOffset(offset, ext, length) {
```
</details>
```
$ ./node-master benchmark/buffers/buffer-slice.js
buffers/buffer-slice.js n=1024 type="fast": 11,632.49754936561
buffers/buffer-slice.js n=1024 type="slow": 11,584.90297078093
$ ./node benchmark/buffers/buffer-slice.js
buffers/buffer-slice.js n=1024 type="fast": 6,675.602412489698
buffers/buffer-slice.js n=1024 type="slow": 6,487.594815897296
```
I'd like to work with the V8 team to resolve the performance problem, and eventually use the language built-in for `Buffer.prototype.slice`.
/cc @bmeurer | buffer,v8 engine,performance | low | Major |
278,740,707 | rust | method-probing machinery can false-positive | [While working on improving method suggestions](https://github.com/rust-lang/rust/issues/42929#issuecomment-345914556), it was discovered that the return value of `probe_for_return_type`—at least, a slightly altered version thereof that passes `ProbeScope::AllTraits` to `probe_op` rather than [`ProbeScope::TraitsInScope` (as on master at issue-filing time)](https://github.com/rust-lang/rust/blob/088f328e/src/librustc_typeck/check/method/probe.rs#L186-L195)—could include methods that don't actually return the desired type. Most notably, this happened with `core::convert::Into::into`.
The root cause _seems_ to be that when we [try to resolve type variables](https://github.com/rust-lang/rust/blob/088f328e/src/librustc_typeck/check/method/probe.rs#L1084), we might [find that the return type of the trait method still needs to be resolved](https://github.com/rust-lang/rust/blob/088f328e/src/librustc/infer/mod.rs#L1282-L1284), and that unresolved type variable is [considered to fulfill the required return type](https://github.com/rust-lang/rust/blob/78fcf3388/src/librustc_typeck/check/method/probe.rs#L1089)?? (I regret my difficulty to provide a better report than this.) | A-diagnostics,T-compiler,C-bug,WG-diagnostics | low | Minor |
278,742,863 | rust | method-probing machinery can false-negative because it doesn't know about associated types | As part of [the endeavor](https://github.com/rust-lang/rust/issues/42929#issuecomment-345914556) that also prompted #46459, it was noticed that we never generated a suggestion for `.to_owned()` even where it would be appropriate. Some strategic logging in `matches_return_type` revealed that the result being returned [by `.can_sub`](https://github.com/rust-lang/rust/blob/16ba459/src/librustc_typeck/check/method/probe.rs#L708) was—
```
Err(
Sorts(
ExpectedFound {
expected: <_ as std::borrow::ToOwned>::Owned,
found: std::string::String
}
)
)
```
But in this case, `ToOwned::Owned` should have [_been_](https://github.com/rust-lang/rust/blob/16ba4591d/src/liballoc/str.rs#L187) `String`.
| C-enhancement,A-diagnostics,T-compiler,S-needs-repro | low | Minor |
278,805,226 | rust | Moving some code around results in linking error with incremental comp | I've had this happen 3 times now today. It's fixed by `cargo clean`, so is not a critical issue, but still is a moderately interesting incremental compilation bug. The change was moving the trait `query_dsl::OffsetDsl` to `query_dsl::methods::OffsetDsl`. The code in question that is failing was importing `OffsetDsl::offset` from `diesel::prelude` instead, the `x.offset` is now resolving to `query_dsl::QueryDsl::offset`, which simply calls `OffsetDsl::offset`, and is exported from prelude.
Anyway, this is the error
<img width="1360" alt="screen shot 2017-12-03 at 11 07 06 am" src="https://user-images.githubusercontent.com/1529387/33528246-9d44600a-d81a-11e7-889c-374ee6b3f195.png"> and the patch that caused it is https://gist.github.com/sgrif/8bb17b2a143d482e060fc3fd08705067
`rustc --version`: rustc 1.24.0-nightly (73bca2b9f 2017-11-28)
| T-compiler,A-incr-comp,C-bug | low | Critical |
278,822,490 | TypeScript | Proposal: support pathof along with current keyof | <!-- SUGGESTIONS: See https://github.com/Microsoft/TypeScript-wiki/blob/master/Writing-Good-Design-Proposals.md -->
## Problem - What are users having difficulty with?
While using popular libraries for their day to day development needs, developers can find them disjoint from typesafe philosophy even if they do have the needed typings. This is true for frequently used libraries such as lodash and immutablejs that access or set properties via paths. It is up to the developers to to preserve typesafety by expressing complex types using the amazing power that typescript gives them. The only obstacle is the absence of a way to express the type that represnts legit object paths for a specific type.
## Current state
We can currently do this only for shallow objects where paths can be simply expressed as the keys of a specific type.
## Example Issue
In an effort to play with stricter typings for immutablejs map I created this tiny project :
https://github.com/agalazis/typed-map/
(as a side note my personal view is that immutable js map is not a conventional map that should be represented with map<keyType, valueType> since it represents a map that matches a specific type rather than just a key,value data structure as demonstarated in `src/examples`)
The type I created was just this (only playing with get and set):
```ts
export type TypedMap<T> = {
get: <K extends keyof T >(k:K) => T[K];
set: <K extends keyof T >(k:K, v:T[K]) => TypedMap<T>;
}
```
Simple enough, leveraging all the expressiveness of typescript.
## Example usage of the proposed solution:
If I could replace keyof with pathof in order to express possible path strings and also used T[P] as the path type I would be able to completely cover this use-case :
```ts
export type TypedMap<T> = {
get: <P extends pathof T >(p:P) => T[P];
set: <P extends pathof T>(p:P, v:T[P]) => TypedMap<T>;
}
```
## Possible usage in the following libraries:
- lodash
- immutable
- ramda
- anything built on top of them
### Why bother since this does not involve facilitating any feature of ES standard?(and is somewhat an unconventional feature)
1. We will be able to perform type-safe updates using existing js immutability libraries similar to what you can achieve in other languages (eg. [skala lense](http://koff.io/posts/292173-lens-in-scala/)).
2. This feature complies with typescript goals:
- Statically identify constructs that are likely to be errors.
- Provide a structuring mechanism for larger pieces of code (the above-mentioned libraries are used every day in big projects imagine the chaos that can be created. Despite exhaustive unit testing, intellisense is a must ;) ).
- Strike a balance between correctness and productivity.
4. This feature improves compile time checks and sticks to the mindset of not having to deal with any runtime functionality
3. There are no side effects in the generated js code
### Alternatives
While digging further into the issue an alternative solution is to be able to spread keyof recursively. This will allow us to be creative and build our own solution as per:
https://github.com/Microsoft/TypeScript/issues/20423#issuecomment-349776005
The drawbacks of the alternative solution if doable are:
- performance
- lack of cyclic reference support
### Implementation Suggestions
The optimal (if implemented in the language) would be to not compute the full nested object paths but only the paths used via pathof ie when declaring something as path of x just accept it as pathof x then when a value is assigned just validate it is pathOf x( the compiler could also have some sort of caching so that it doesn't recalculate paths).
This will also solve the issue with cyclic references since paths will be finite anw.
| Suggestion,Awaiting More Feedback | medium | Critical |
278,835,863 | pytorch | Raise an error when using magma built against wrong version of cuda | Previous instances of this: https://github.com/pytorch/pytorch/issues/3018
I myself was affected by this bug when a server I was working on upgraded from cuda 8 to cuda 9. I dutifully rebuilt PyTorch but forgot to uninstall magma-cuda80: instant hang on CUDA initialization. | module: binaries,module: build,triaged | low | Critical |
278,959,528 | rust | Proc macro hygiene regression | Something in the range https://github.com/rust-lang/rust/compare/bb42071f6...f9b0897c5, likely https://github.com/rust-lang/rust/pull/46343 (CC @jseyfried), broke Servo.
We have a `#[dom_struct]` attribute implemented in a `dom_struct` crate like this:
```rust
#[proc_macro_attribute]
pub fn dom_struct(args: TokenStream, input: TokenStream) -> TokenStream {
if !args.is_empty() {
panic!("#[dom_struct] takes no arguments");
}
let attributes = quote! {
#[derive(DenyPublicFields, DomObject, JSTraceable, MallocSizeOf)]
#[must_root]
#[repr(C)]
};
iter::once(attributes).chain(iter::once(input)).collect()
}
```
Each of the derives is defined in a respective crate. The `script` crate depends on all of these crates and uses `#[dom_struct]`. Some of the derives generate code that reference items defined in `script`. For example, `#[derive(DomObject)]` implements the `script::dom::bindings::refector::DomObject` trait.
Since rustc 1.24.0-nightly (f9b0897c5 2017-12-02), every use of `#[dom_struct]` fails with:
```rust
error[E0433]: failed to resolve. Could not find `js` in `{{root}}`
--> components/script/dom/attr.rs:28:1
|
28 | #[dom_struct]
| ^^^^^^^^^^^^^ Could not find `js` in `{{root}}`
error[E0433]: failed to resolve. Could not find `dom` in `{{root}}`
--> components/script/dom/attr.rs:28:1
|
28 | #[dom_struct]
| ^^^^^^^^^^^^^ Could not find `dom` in `{{root}}`
error[E0433]: failed to resolve. Could not find `js` in `{{root}}`
--> components/script/dom/attr.rs:28:1
|
28 | #[dom_struct]
| ^^^^^^^^^^^^^ Could not find `js` in `{{root}}`
error[E0433]: failed to resolve. Could not find `malloc_size_of` in `{{root}}`
--> components/script/dom/attr.rs:28:1
|
28 | #[dom_struct]
| ^^^^^^^^^^^^^ Could not find `malloc_size_of` in `{{root}}`
```
I suppose that these errors come from code generated by the derives, and that `{{root}}` refers to the root of the `dom_struct` crate where the `#[derive(…)]` tokens come from. Indeed, the `js` and `dom` are not an cannot be available there, they’re in the `script` crate which depends on `dom_struct`.
We can work around this by erasing hygiene data in the `#[derive(…)]` tokens:
```diff
--- a/components/dom_struct/lib.rs
+++ b/components/dom_struct/lib.rs
@@ -17,7 +17,9 @@ pub fn dom_struct(args: TokenStream, input: TokenStream) -> TokenStream {
let attributes = quote! {
#[derive(DenyPublicFields, DomObject, JSTraceable, MallocSizeOf)]
#[must_root]
#[repr(C)]
};
+ let attributes = attributes.to_string().parse().unwrap();
iter::once(attributes).chain(iter::once(input)).collect()
}
```
… but this seems like a valid pattern that shouldn’t necessitate such hacks. | P-medium,A-macros,T-compiler,regression-from-stable-to-stable,C-bug,A-hygiene | medium | Critical |
278,993,779 | create-react-app | Symlink behaviour | If I add a symlink in my src directory to another directory, and then include files from that path, create-react-app gives an error:
> Module parse failed: Unexpected token
> You may need an appropriate loader to handle this file type.
I assume create-react-app wants to have this import already built/transpiled. I would expect this to be the case for imports outside the src directory (node_modules for example). But since the symlink resides in the src directory, I would assume create-react-app would fetch these files as if they were truly in src directory.
Is this a bug, or expected behaviour? It makes it really hard to extract common components.
| issue: proposal | high | Critical |
279,046,200 | rust | Rustc segfaults with -C passes=livestacks | How to reproduce:
```
mkdir /tmp/repro
cd /tmp/repro
cargo init --bin
mkdir .cargo
echo '[build]\nrustflags = ["-C", "passes=livestacks"]' > .cargo/config
cargo build --verbose
```
Output:
```
Compiling repro v0.1.0 (file:///tmp/repro)
Running `rustc --crate-name repro src/main.rs --crate-type bin --emit=dep-info,link -C debuginfo=2 -C metadata=10c9440624901274 -C extra-filename=-10c9440624901274 --out-dir /tmp/repro/target/debug/deps -L dependency=/tmp/repro/target/debug/deps -C passes=livestacks`
error: Could not compile `repro`.
Caused by:
process didn't exit successfully: `rustc --crate-name repro src/main.rs --crate-type bin --emit=dep-info,link -C debuginfo=2 -C metadata=10c9440624901274 -C extra-filename=-10c9440624901274 --out-dir /tmp/repro/target/debug/deps -L dependency=/tmp/repro/target/debug/deps -C passes=livestacks` (signal: 11, SIGSEGV: invalid memory reference)
```
The `livestacks` pass is listed by `rustc -C passes=list`.
Reproduces on 1.21 and nightly:
```
rustc --version
rustc 1.21.0 (3b72af97e 2017-10-09)
rustc +nightly --version
rustc 1.24.0-nightly (1956d5535 2017-12-03)
``` | I-crash,A-LLVM,T-compiler,C-bug | low | Critical |
279,056,684 | godot | Configure Snap menu should use vector input for grid, and number input for rotation | **Operating system or device, Godot version, GPU Model and driver (if graphics related):**
Godot 3.0 Beta 1
**Issue description:**
Currently the configure snap menu uses two input fields for grid offset and grid size. I think it would be better if it used the vector input like node properties use. The rotation inputs should also use the same number input that the node properties have, with a range of 0 to 360. The "px" and "deg" should probably be labels outside of the number boxes, not inside them. These changes would make setting the numbers quicker and easier.

| enhancement,topic:editor | low | Major |
279,064,744 | go | x/net/publicsuffix: ICANN flag returned not aligned with public suffix location in the list | Please answer these questions before submitting your issue. Thanks!
### What version of Go are you using (`go version`)?
go version go1.9.2
### Does this issue reproduce with the latest release?
Yes
### What operating system and processor architecture are you using (`go env`)?
goos: darwin
goarch: amd64
### What did you do?
Call PublicSuffix with input domain "transurl.be"
https://play.golang.org/p/SLdVLEzpk4
### What did you expect to see?
ICANN flag set to true
List contains "be" within the ICANN delimiters:
// be : https://en.wikipedia.org/wiki/.be
// Confirmed by registry <[email protected]> 2008-06-08
be
ac.be
and "*.transurl.be" outside.
// TransIP : htts://www.transip.nl
// Submitted by Rory Breuk <[email protected]>
*.transurl.be
*.transurl.eu
*.transurl.nl
### What did you see instead?
ICANN flag set to false
I am not sure if this behaviour is expected. Should transurl.be match *.transurl.be, given it doesn't have a 3rd level domain?
Also, since the public suffix domain returned is "be" , I would expect for that rule to be the one being matched, and therefore having ICANN set to true.
There are more occurrences of this scenario, such as "0emm.com".
| NeedsInvestigation | low | Major |
279,126,198 | rust | Unreachable code warning for std::unreachable! | Presently, rustc will emit a warning when it detects unreachable code. Ordinarily, this is great, but in the case of `std::unreachable!`, it isn't particularly helpful.
For example, consider the following code:
```
#![deny(unreachable_code)]
fn main() {
return;
unreachable!();
}
```
([Playground link](https://play.rust-lang.org/?gist=5fd1d9f2b23cc75b8419120513406274&version=stable))
This results in:
```
error: unreachable statement
--> src/main.rs:6:5
|
6 | unreachable!();
| ^^^^^^^^^^^^^^^
|
note: lint level defined here
--> src/main.rs:1:9
|
1 | #![deny(unreachable_code)]
| ^^^^^^^^^^^^^^^^
= note: this error originates in a macro outside of the current crate
```
In this case the warning isn't particularly helpful given that I've explicitly marked the code as unreachable. It seems like it would be reasonable to avoid emitting a warning in this case.
In particular, this is problematic because `#![deny(unreachable_code)]` is implied by `#![deny(warnings)]`. I'm working in a large codebase that makes heavy use of `#![deny(warnings)]`. As a result, I'm not able to use `std::unreachable!()` without explicitly allowing unreachable code, which isn't desirable.
Oftentimes I'd like to use `std::unreachable!()` in places where unreachability is statically provable, but not intuitively obvious to a human reading the code. For now I've settled on just using comments instead.
| C-enhancement,A-lints | low | Critical |
279,200,892 | flutter | Secondary isolates should get dart:ui bindings. | Secondary isolates currently do not have access to any of the window bindings that back `dart:ui`. Clients that attempt to use parts of the Flutter API that depend on the same run into exceptions because of this.
It is theoretically safe for secondary isolates to get some `dart:ui` bindings already. However, there are some dart callbacks that are made after traversing threads. This includes scheduling frames in response to vsync pulses. If a secondary thread were to get access to the Dart UI bindings, it would be able to schedule frames that the root isolate would then have to respond to. So making all secondary isolates get the UI binding is a no-go.
We can attempt to address this issue in one of two ways:
* Wait for the shell refactor to land. This allows each isolate to have its own set of task runners. Frame scheduling attempted by the secondary isolates would be a no-op because its platform and GPU task runners are null.
* Design a `dart:ui` lite variant of bindings that are safely accessible by secondary isolates.
* Make the Flutter API resilient to uninitialized items in the `dart:ui` and don't support UI bindings on secondary threads at all. | engine,dependency: dart,c: proposal,P2,team-engine,triaged-engine | low | Major |
279,331,643 | angular | [Animations] params not evaluated when transitioning to void state (e.g. ngIf :leave) | ## I'm submitting a...
<pre><code>
[ ] Regression (a behavior that used to work and stopped working in a new release)
[x] Bug report
[ ] Feature request
[ ] Documentation issue or request
[ ] Support request
</code></pre>
## Current behavior
I want to animate an element which is being added and removed with *ngIf. I need to pass a parameter to the animation at run-time, which I am doing like this:
```HTML
<!-- app.component.html -->
<div *ngIf="show" [@myTrigger]="triggerParams">
Hello.
</div>
```
```TypeScript
@Component({
selector: 'my-app',
templateUrl: `./app.component.html`,
animations: [
trigger('myTrigger', [
state('*', style({ opacity: '{{ opacity }}' }), { params: { opacity: 0 } }),
state('void', style({ opacity: '{{ opacity }}' }), { params: { opacity: 0 } }),
transition('void => *', animate('1000ms')),
transition('* => void', animate('1000ms'))
]),
]
})
export class AppComponent {
show = false;
get triggerParams(): any {
return {
value: '',
params: {
opacity: this.show ? 1 : 0
}
};
}
}
```
Currently, the `triggerParams` getter is being evaluated during the transition from `void => *`, but not the other way around, with the result that the div fades in, but does not fade out.
## Expected behavior
I would expect the `triggerParams` getter to be evaluated also when the div transitions from `* => void` so that the param is set to `{ opacity: 0 }` and it fades out.
## Minimal reproduction of the problem with instructions
https://stackblitz.com/edit/repro-for-20796?file=app/app.component.ts
## What is the motivation / use case for changing the behavior?
So that I can animate *ngIf with dynamic params.
## Environment
<pre><code>
Angular version: 4.4.6, 5.0.5, 5.1.0-rc.1 (tested all 3)
Browser:
- [x] Chrome (desktop) version 62
For Tooling issues:
- Node version: 8.9.1
- Platform: Windows 10
</code></pre>
| type: bug/fix,area: animations,freq2: medium,P3 | low | Critical |
279,334,741 | angular | feat: allow HTTP param encoder to vary for arrays | <!--
PLEASE HELP US PROCESS GITHUB ISSUES FASTER BY PROVIDING THE FOLLOWING INFORMATION.
ISSUES MISSING IMPORTANT INFORMATION MAY BE CLOSED WITHOUT INVESTIGATION.
-->
## I'm submitting a...
<!-- Check one of the following options with "x" -->
<pre><code>[x] Bug report
</code></pre>
## Current behavior
When I send url data parameter which is an array on a GET request, only the first element is sended.
```
let testParams = {key: 'value', array: ['v1','v2']};
this.http.get(`url`, {params: testParams}).subscribe();
```
> http://127.0.0.1/url?key=value&array=v1
## Expected behavior
The whole array parameters must be send like that:
> http://127.0.0.1/url?key=value&array[]=v1&array[]=v2
## Environment
<pre><code>
Angular version: 5.0.2
<!-- Check whether this is still an issue in the most recent Angular version -->
Browser:
- [x] Chrome (desktop) version 62.0.3202.94
For Tooling issues:
- Node version: 8.9.1
- Platform: win32 x64
</code></pre>
| feature,freq1: low,area: common/http,P3,feature: under consideration | low | Critical |
279,346,088 | angular | Query through components are not working | ## I'm submitting a...
<pre><code>[ ] Regression (a behavior that used to work and stopped working in a new release)
[x] Bug report
[ ] Feature request
[ ] Documentation issue or request
[ ] Support request</code></pre>
## Current behavior
Cannot query for a leave animation which is inside a child component.
## Expected behavior
The parent animation should be able to query for leave animations which are multiple level below in the component tree.
## Minimal reproduction of the problem with instructions
https://stackblitz.com/edit/angular-nfr1yb
Here if the `ParentComponent` gets removed it does not wait for the `ChildComponent` leave animation.
## Environment
<pre><code>Angular version: 7.1.0</code></pre> | type: bug/fix,area: animations,freq3: high,P3 | low | Critical |
279,357,871 | vue | Establish a standard way to document component and its props | ### What problem does this feature solve?
https://github.com/vuejs/vetur/issues/276
Currently, Vetur offers auto-completion & hover info for custom components defined in ElementUI, OnsenUI and Bootstrap-Vue. However, hand-maintaining such json files seem to be a lot of work. Also not co-locating the doc and the component definition can make updating component challenging.
Helper-json repos at:
- https://github.com/ElementUI/element-helper-json
- https://www.npmjs.com/package/vue-onsenui-helper-json
- https://github.com/bootstrap-vue/bootstrap-vue-helper-json
This feature makes it possible to write the doc in the SFC / js component file, and auto-generate a helper json that can be used for enhancing editing experience or auto-generating API / doc website.
### What does the proposed API look like?
Two more optional attributes on the default export:
```js
export default {
name: 'v-card',
description: 'A card component',
props: ['width', 'height'],
propsDescription: [
'width of the rendered card component',
'height of the rendered card component'
]
}
```
I was thinking maybe using a custom block for it, but then that only applies to SFC, not js components. jsdoc might be another option.
Other ideas welcome.
Another idea is similar to the `typings` in package.json, have a `vueTypings` for component libraries. It'll point to the generated helper-json file and editors could pick it up to enhance editing experience.
/cc
@Leopoldthecoder for ElementUI
@masahirotanaka for OnsenUI
@pi0 for Bootstrap-Vue
@rstoenescu for Quasar
@johnleider for Vuetify
Would you be interested in using this feature in the Vue component libraries that you are maintaining? Would you be interested in helping spec'ing a format for the generated json file and the editing experiences that should be enabled by using that file?
<!-- generated by vue-issues. DO NOT REMOVE --> | discussion | high | Critical |
279,451,896 | go | x/text: FAIL: TestCountMallocs | Hello !
### What version of Go are you using (`go version`)? 1.8
### Does this issue reproduce with the latest release? version 0.1.0
### What did you do?
I try to test message in the project github.com/golang/text/ in version 0.1.0 it fails with
--- FAIL: TestCountMallocs (0.00s)
fmt_test.go:1443: Sprintf("%x"): got 3 allocs, want <=2
fmt_test.go:1443: Sprintf("%s"): got 3 allocs, want <=2
fmt_test.go:1443: Sprintf("%x %x"): got 4 allocs, want <=3
fmt_test.go:1443: Sprintf("%g"): got 5 allocs, want <=2
fmt_test.go:1443: Fprintf(buf, "%s"): got 2 allocs, want <=1
fmt_test.go:1443: Fprintf(buf, "%x %x %x"): got 1 allocs, want <=0
FAIL
exit status 1
FAIL golang.org/x/text/message 0.023s
Thank you for your help
| NeedsInvestigation | low | Minor |
279,552,440 | go | cmd/compile: slicing can be improved on ARM | This code:
```go
func slice(s []int64, idx int) []int {
return s[idx:]
}
```
generates this code on ARM:
```
CMP R0, R7 // bound check
B.GE panic
SUB R7, R0, R0 // len
SUB R7, R4, R4 // cap
RSB $0, R4, R9 // mask(cap)
ASR $31, R9, R9 // mask(cap)
AND R7<<$3, R9, R9 // delta&mask(cap)
ADD R5, R9, R5 // ptr+delta&mask(cap)
```
but it could be optimized this way:
```
SUBS R7, R0, R0
B.LS panic
SUBS R7, R4, R4
ADD.NE R7<<$3, R9, R5
```
which is much shorter and faster.
| Performance,compiler/runtime | low | Major |
279,563,738 | angular | [Animations, Angular 5] Nested [@triggerName] fires when parent trigger is executed. Animation executes before its turn | <!--
PLEASE HELP US PROCESS GITHUB ISSUES FASTER BY PROVIDING THE FOLLOWING INFORMATION.
ISSUES MISSING IMPORTANT INFORMATION MAY BE CLOSED WITHOUT INVESTIGATION.
-->
## I'm submitting a...
<!-- Check one of the following options with "x" -->
<pre><code>
[ X ] Regression (a behavior that used to work and stopped working in a new release)
[ ] Bug report <!-- Please search GitHub for a similar issue or PR before submitting -->
[ ] Feature request
[ ] Documentation issue or request
[ ] Support request => Please do not submit support request here, instead see https://github.com/angular/angular/blob/master/CONTRIBUTING.md#question
</code></pre>
## Current behavior
The issue in a nutshell: A nested @trigger animates when the parent animates, ignoring it's delay.
In the following SVG ( a lock), I have added animated parts to move it's elements and change the colour during the animation.
Right now, when the top lock part close (moves down) the child animation on the path fires too, while I have programmed it to wait 900ms before executing.
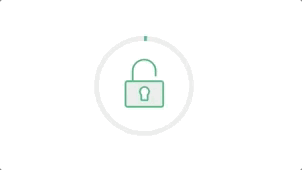
As you can see in the GIF, the top lock part turns red at the same time it slides down. Then the rest of the lock turns red later. They all use the same `@fillState` trigger, however the top part runs at the same time as it `@lockState` is executed.
It needs to 1) close the lock, 2) and then change colour. Not at the same time.
This was working in Angular 4.4.2
```
<svg class="icon-lock-open" viewBox="0 0 46 58" version="1.1" xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink">
<defs>
<polygon id="path-1" points="0.412 0.329 29.234 0.329 29.234 25.9964355 0.412 25.9964355"></polygon>
<polygon id="path-3" points="45.762 15.941 45.762 31.846 0 31.846 0 0.036 45.762 0.036"></polygon>
</defs>
<g stroke="none" stroke-width="1" fill="none" fill-rule="evenodd">
<g>
<g transform="translate(7.830000, 0.000000)" [@lockState]="state">
<path id="lock-top" fill="#38B872" [@fillState]="state" fill-rule="nonzero" d="M6.1862"></path>
</g>
<path id="lock-base-overlay" fill="#E8E9E8" [@lockFillState]="state" fill-rule="nonzero" d="M27.02"></path>
<g transform="translate(0.000000, 25.230000)">
<path id="lock-base" fill="#38B872" [@fillState]="state" fill-rule="nonzero" d="M3.255,"></path>
</g>
<path id="lock-key-hole" fill="#38B87`2" [@fillState]="state" fill-rule="nonzero" d="M26.8"></path>
</g>
</g>
</svg>
```
```
animations: [
trigger( 'lockState', [
state( 'open', style( {
transform: 'translate3d( 7.83px, 0, 0)',
// transform: 'scale(1)',
} ) ),
state( 'locked', style( {
transform: 'translate3d( 7.83px, 5px, 0)',
} ) ),
transition( 'open => locked', animate( '100ms 500ms linear' ) ),
// transition( 'locked => open', animate( '100ms ease-out' ) ),
] ),
// lock part that turns from green to white
//
trigger( 'fillState', [
state( 'open', style( {
fill: '#38B872',
} ) ),
state( 'locked', style( {
fill: 'red',
} ) ),
transition( 'open => locked', animate( '300ms 900ms linear' ) ),
] ),
.... ]
```
## Expected behavior
The @lockState trigger animates first, closing the lock, then the @fillState animation executes (since it is delayed by 900ms). The lock turns from green into red (normally it would be white colour, just for testing).
## Minimal reproduction of the problem with instructions
<!--
For bug reports please provide the *STEPS TO REPRODUCE* and if possible a *MINIMAL DEMO* of the problem via
https://plnkr.co or similar (you can use this template as a starting point: http://plnkr.co/edit/tpl:AvJOMERrnz94ekVua0u5).
-->
I will try adding a plunker later.
## What is the motivation / use case for changing the behavior?
Well, I had the animation working in Angular 4.4.2. Now that I have migrated to Angular 5.0.5 the animation is working differently. I can try to create separate @triggerStates or play with groups, but I want to make sure that what I am seeing is not a bug.
## Environment
<pre><code>
Angular version: 5.0.5
<!-- Check whether this is still an issue in the most recent Angular version -->
Browser:
- [ X ] Chrome (desktop) version 62.0.3202.94
- [ ] Chrome (Android) version XX
- [ ] Chrome (iOS) version XX
- [ ] Firefox version XX
- [ ] Safari (desktop) version XX
- [ ] Safari (iOS) version XX
- [ ] IE version XX
- [ ] Edge version XX
For Tooling issues:
- Node version: XX <!-- run `node --version` -->
- Platform: <!-- Mac, Linux, Windows -->
Others:
<!-- Anything else relevant? Operating system version, IDE, package manager, HTTP server, ... -->
</code></pre>
| type: bug/fix,area: animations,freq1: low,P3 | low | Critical |
279,588,856 | go | net/http: local IP address change caused timeouts for outgoing HTTPS requests | Using Go 1.9.2 on linux amd64.
Using the net/http client on a device whose IP address changes while the program is running, we started getting timeouts for HTTPS requests. To troubleshoot, we set up a DHCP server with very short leases and a minimal program that made requests every 10s and were able to reproduce the problem. We used a client with a transport based on http.DefaultTransport and a timeout (you can see it below).
I found I had to set DisableKeepAlives on the transport or set the IdleConnTimeout shorter than the frequency of our requests, or HTTPS requests would fail consistently after the IP address changed.
The following shows one run of the program. It was compiled with Go 1.9.2. The four routines are: HTTP with keep alive, HTTPS with keep alive disabled, HTTPS with keep alive, and HTTPS with the default http.Client (no timeout, keep alive enabled).
After the IP changes at about 4:04:10, you can see both the HTTP and HTTPS with keep alive fail. HTTP recovers (it isn't worth caching?), but HTTPS keeps timing out. As expected, HTTPS with the default client never times out or recovers.
I'll include the code used to generate this output below. But it's not trivial to reproduce because you need a DHCP server with a short lease and configurable static IPs.
In the short-term, we're just going to disable keep alives for our transports; but I'm reporting this because graceful IP renewal handling seems something Go should have.
---
```
2017/12/05 04:03:46 HTTP / keep alive 204
2017/12/05 04:03:47 HTTPS / default 204
2017/12/05 04:03:47 HTTPS / keep alive 204
2017/12/05 04:03:47 HTTPS / disabled 204
2017/12/05 04:03:56 HTTP / keep alive 204
2017/12/05 04:03:57 HTTPS / default 204
2017/12/05 04:03:57 HTTPS / keep alive 204
2017/12/05 04:03:57 HTTPS / disabled 204
2017/12/05 04:04:06 HTTP / keep alive 204
2017/12/05 04:04:07 HTTPS / default 204
2017/12/05 04:04:07 HTTPS / keep alive 204
2017/12/05 04:04:07 HTTPS / disabled 204
2017/12/05 04:04:17 HTTPS / disabled 204
2017/12/05 04:04:27 HTTPS / disabled 204
2017/12/05 04:04:36 HTTP / keep alive Get http://www.google.com/generate_204: net/http: request canceled (Client.Timeout exceeded while awaiting headers)
2017/12/05 04:04:37 HTTPS / keep alive Get https://www.google.com/generate_204: net/http: request canceled (Client.Timeout exceeded while awaiting headers)
2017/12/05 04:04:37 HTTPS / disabled 204
2017/12/05 04:04:47 HTTP / keep alive 204
2017/12/05 04:04:47 HTTPS / disabled 204
2017/12/05 04:04:57 HTTP / keep alive 204
2017/12/05 04:04:57 HTTPS / disabled 204
2017/12/05 04:05:07 HTTPS / keep alive Get https://www.google.com/generate_204: net/http: request canceled (Client.Timeout exceeded while awaiting headers)
2017/12/05 04:05:07 HTTP / keep alive 204
2017/12/05 04:05:08 HTTPS / disabled 204
2017/12/05 04:05:17 HTTP / keep alive 204
2017/12/05 04:05:18 HTTPS / disabled 204
2017/12/05 04:05:27 HTTP / keep alive 204
2017/12/05 04:05:28 HTTPS / disabled 204
2017/12/05 04:05:37 HTTPS / keep alive Get https://www.google.com/generate_204: net/http: request canceled (Client.Timeout exceeded while awaiting headers)
2017/12/05 04:05:37 HTTP / keep alive 204
2017/12/05 04:05:38 HTTPS / disabled 204
2017/12/05 04:05:47 HTTP / keep alive 204
2017/12/05 04:05:48 HTTPS / disabled 204
2017/12/05 04:05:57 HTTP / keep alive 204
2017/12/05 04:05:58 HTTPS / disabled 204
2017/12/05 04:06:07 HTTPS / keep alive Get https://www.google.com/generate_204: net/http: request canceled (Client.Timeout exceeded while awaiting headers)
2017/12/05 04:06:07 HTTP / keep alive 204
2017/12/05 04:06:08 HTTPS / disabled 204
2017/12/05 04:06:17 HTTP / keep alive 204
2017/12/05 04:06:18 HTTPS / disabled 204
2017/12/05 04:06:27 HTTP / keep alive 204
2017/12/05 04:06:28 HTTPS / disabled 204
2017/12/05 04:06:37 HTTPS / keep alive Get https://www.google.com/generate_204: net/http: request canceled (Client.Timeout exceeded while awaiting headers)
2017/12/05 04:06:37 HTTP / keep alive 204
2017/12/05 04:06:38 HTTPS / disabled 204
```
----
```Go
package main
import (
"log"
"net"
"net/http"
"time"
)
func main() {
clients := []struct {
label string
client *http.Client
url string
}{
{"HTTPS / disabled", client(true), "https://www.google.com/generate_204"},
{"HTTPS / keep alive", client(false), "https://www.google.com/generate_204"},
{"HTTPS / default", http.DefaultClient, "https://www.google.com/generate_204"},
{"HTTP / keep alive", client(false), "http://www.google.com/generate_204"},
}
var i int
for i = 0; i < len(clients)-1; i++ {
go makeRequests(clients[i].label, clients[i].client, clients[i].url)
}
makeRequests(clients[i].label, clients[i].client, clients[i].url)
}
func client(dka bool) *http.Client {
return &http.Client{
Timeout: 20 * time.Second,
Transport: &http.Transport{
DisableKeepAlives: dka,
Proxy: http.ProxyFromEnvironment,
DialContext: (&net.Dialer{
Timeout: 30 * time.Second,
KeepAlive: 30 * time.Second,
DualStack: true,
}).DialContext,
MaxIdleConns: 100,
IdleConnTimeout: 50 * time.Second,
TLSHandshakeTimeout: 10 * time.Second,
ExpectContinueTimeout: 1 * time.Second,
},
}
}
func makeRequests(label string, client *http.Client, url string) error {
for {
sc, err := makeRequest(client, url)
if err != nil {
log.Printf(" %-20s %v\n", label, err)
} else {
log.Printf(" %-20s %d\n", label, sc)
}
time.Sleep(10 * time.Second)
}
}
func makeRequest(client *http.Client, url string) (int, error) {
req, err := http.NewRequest("GET", url, nil)
if err != nil {
return 0, err
}
resp, err := client.Do(req)
if err != nil {
return 0, err
}
defer resp.Body.Close()
return resp.StatusCode, nil
}
``` | NeedsInvestigation | low | Critical |
279,595,032 | youtube-dl | Youtube download error - <urlopen error [Errno 22] Invalid argument> | ## Please follow the guide below
- You will be asked some questions and requested to provide some information, please read them **carefully** and answer honestly
- Put an `x` into all the boxes [ ] relevant to your *issue* (like this: `[x]`)
- Use the *Preview* tab to see what your issue will actually look like
---
### Make sure you are using the *latest* version: run `youtube-dl --version` and ensure your version is *2017.12.02*. If it's not, read [this FAQ entry](https://github.com/rg3/youtube-dl/blob/master/README.md#how-do-i-update-youtube-dl) and update. Issues with outdated version will be rejected.
- [x] I've **verified** and **I assure** that I'm running youtube-dl **2017.12.02**
### Before submitting an *issue* make sure you have:
- [x] At least skimmed through the [README](https://github.com/rg3/youtube-dl/blob/master/README.md), **most notably** the [FAQ](https://github.com/rg3/youtube-dl#faq) and [BUGS](https://github.com/rg3/youtube-dl#bugs) sections
- [x] [Searched](https://github.com/rg3/youtube-dl/search?type=Issues) the bugtracker for similar issues including closed ones
### What is the purpose of your *issue*?
- [x] Bug report (encountered problems with youtube-dl)
- [ ] Site support request (request for adding support for a new site)
- [ ] Feature request (request for a new functionality)
- [ ] Question
- [ ] Other
---
```
[debug] System config: []
[debug] User config: []
[debug] Custom config: []
[debug] Command-line args: [u'https://www.youtube.com/watch?v=ejPe2eYhjR4', u'-v']
[debug] Encodings: locale UTF-8, fs utf-8, out UTF-8, pref UTF-8
[debug] youtube-dl version 2017.12.02
[debug] Python version 2.7.14 - Darwin-17.2.0-x86_64-i386-64bit
[debug] exe versions: ffmpeg 3.4, ffprobe 3.4
[debug] Proxy map: {}
[youtube] ejPe2eYhjR4: Downloading webpage
[youtube] ejPe2eYhjR4: Downloading video info webpage
[youtube] ejPe2eYhjR4: Extracting video information
[youtube] ejPe2eYhjR4: Downloading MPD manifest
[debug] Default format spec: bestvideo+bestaudio/best
[debug] Invoking downloader on u'https://r1---sn-nu5gi0c-npok.googlevideo.com/videoplayback?id=7a33ded9e6218d1e&itag=137&source=youtube&requiressl=yes&ei=V0knWt3fBIbsowOg1IfgCg&mn=sn-nu5gi0c-npok&mm=31&ms=au&mv=u&pl=43&ratebypass=yes&mime=video/mp4&gir=yes&clen=92139427&lmt=1512416704858018&dur=195.153&signature=32
9F0B18B27DB4AA9F6F9C8BF58835F8984356C8.FBE391BCB4F0C2A7BEDF79D7635AAD71E92886&mt=1512523703&key=dg_yt0&ip=2406:3000:11:1022:9d80:1845:6e37:751d&ipbits=0&expire=1512545719&sparams=ip,ipbits,expire,id,itag,source,requiressl,ei,mn,mm,ms,mv,pl,ratebypass,mime,gir,clen,lmt,dur'
ERROR: unable to download video data: <urlopen error [Errno 22] Invalid argument>
Traceback (most recent call last):
File "/usr/local/lib/python2.7/site-packages/youtube_dl/YoutubeDL.py", line 1887, in process_info
partial_success = dl(fname, new_info)
File "/usr/local/lib/python2.7/site-packages/youtube_dl/YoutubeDL.py", line 1832, in dl
return fd.download(name, info)
File "/usr/local/lib/python2.7/site-packages/youtube_dl/downloader/common.py", line 361, in download
return self.real_download(filename, info_dict)
File "/usr/local/lib/python2.7/site-packages/youtube_dl/downloader/http.py", line 286, in real_download
establish_connection()
File "/usr/local/lib/python2.7/site-packages/youtube_dl/downloader/http.py", line 74, in establish_connection
ctx.data = self.ydl.urlopen(request)
File "/usr/local/lib/python2.7/site-packages/youtube_dl/YoutubeDL.py", line 2196, in urlopen
return self._opener.open(req, timeout=self._socket_timeout)
File "/usr/local/Cellar/python/2.7.14/Frameworks/Python.framework/Versions/2.7/lib/python2.7/urllib2.py", line 429, in open
response = self._open(req, data)
File "/usr/local/Cellar/python/2.7.14/Frameworks/Python.framework/Versions/2.7/lib/python2.7/urllib2.py", line 447, in _open
'_open', req)
File "/usr/local/Cellar/python/2.7.14/Frameworks/Python.framework/Versions/2.7/lib/python2.7/urllib2.py", line 407, in _call_chain
result = func(*args)
File "/usr/local/lib/python2.7/site-packages/youtube_dl/utils.py", line 1086, in https_open
req, **kwargs)
File "/usr/local/Cellar/python/2.7.14/Frameworks/Python.framework/Versions/2.7/lib/python2.7/urllib2.py", line 1198, in do_open
raise URLError(err)
URLError: <urlopen error [Errno 22] Invalid argument>
```
For completeness, I tested the URL with `curl`
```
$ curl -svo v "https://r1---sn-nu5gi0c-npok.googlevideo.com/videoplayback?id=7a33ded9e6218d1e&itag=137&source=youtube&requiressl=yes&ei=V0knWt3fBIbsowOg1IfgCg&mn=sn-nu5gi0c-npok&mm=31&ms=au&mv=u&pl=43&ratebypass=yes&mime=video/mp4&gir=yes&clen=92139427&lmt=1512416704858018&dur=195.153&signature=329F0B18B27DB4AA9F6F9C8BF58835F8984356C8.FBE391BCB4F0C2A7BEDF79D7635AAD71E92886&mt=1512523703&key=dg_yt0&ip=2406:3000:11:1022:9d80:1845:6e37:751d&ipbits=0&expire=1512545719&sparams=ip,ipbits,expire,id,itag,source,requiressl,ei,mn,mm,ms,mv,pl,ratebypass,mime,gir,clen,lmt,dur"
* Trying 2406:3001:22:1::c...
* TCP_NODELAY set
* Trying 203.116.189.12...
* TCP_NODELAY set
* Connected to r1---sn-nu5gi0c-npok.googlevideo.com (203.116.189.12) port 443 (#0)
* ALPN, offering h2
* ALPN, offering http/1.1
* Cipher selection: ALL:!EXPORT:!EXPORT40:!EXPORT56:!aNULL:!LOW:!RC4:@STRENGTH
* successfully set certificate verify locations:
* CAfile: /usr/local/etc/openssl/cert.pem
CApath: /usr/local/etc/openssl/certs
* TLSv1.2 (OUT), TLS header, Certificate Status (22):
} [5 bytes data]
* TLSv1.2 (OUT), TLS handshake, Client hello (1):
} [512 bytes data]
* TLSv1.2 (IN), TLS handshake, Server hello (2):
{ [87 bytes data]
* TLSv1.2 (IN), TLS handshake, Certificate (11):
{ [3297 bytes data]
* TLSv1.2 (IN), TLS handshake, Server key exchange (12):
{ [333 bytes data]
* TLSv1.2 (IN), TLS handshake, Server finished (14):
{ [4 bytes data]
* TLSv1.2 (OUT), TLS handshake, Client key exchange (16):
} [70 bytes data]
* TLSv1.2 (OUT), TLS change cipher, Client hello (1):
} [1 bytes data]
* TLSv1.2 (OUT), TLS handshake, Finished (20):
} [16 bytes data]
* TLSv1.2 (IN), TLS change cipher, Client hello (1):
{ [1 bytes data]
* TLSv1.2 (IN), TLS handshake, Finished (20):
{ [16 bytes data]
* SSL connection using TLSv1.2 / ECDHE-RSA-AES128-GCM-SHA256
* ALPN, server did not agree to a protocol
* Server certificate:
* subject: C=US; ST=California; L=Mountain View; O=Google Inc; CN=*.googlevideo.com
* start date: Nov 16 20:14:00 2017 GMT
* expire date: Feb 8 20:14:00 2018 GMT
* subjectAltName: host "r1---sn-nu5gi0c-npok.googlevideo.com" matched cert's "*.googlevideo.com"
* issuer: C=US; O=Google Inc; CN=Google Internet Authority G2
* SSL certificate verify ok.
} [5 bytes data]
> GET /videoplayback?id=7a33ded9e6218d1e&itag=137&source=youtube&requiressl=yes&ei=V0knWt3fBIbsowOg1IfgCg&mn=sn-nu5gi0c-npok&mm=31&ms=au&mv=u&pl=43&ratebypass=yes&mime=video/mp4&gir=yes&clen=92139427&lmt=1512416704858018&dur=195.153&signature=329F0B18B27DB4AA9F6F9C8BF58835F8984356C8.FBE391BCB4F0C2A7BEDF79D7635AAD71E92886&mt=1512523703&key=dg_yt0&ip=2406:3000:11:1022:9d80:1845:6e37:751d&ipbits=0&expire=1512545719&sparams=ip,ipbits,expire,id,itag,source,requiressl,ei,mn,mm,ms,mv,pl,ratebypass,mime,gir,clen,lmt,dur HTTP/1.1
> Host: r1---sn-nu5gi0c-npok.googlevideo.com
> User-Agent: curl/7.57.0
> Accept: */*
>
{ [5 bytes data]
< HTTP/1.1 200 OK
< Last-Modified: Mon, 04 Dec 2017 19:45:04 GMT
< Content-Type: video/mp4
< Date: Wed, 06 Dec 2017 01:36:30 GMT
< Expires: Wed, 06 Dec 2017 01:36:30 GMT
< Cache-Control: private, max-age=21229
< Accept-Ranges: bytes
< Content-Length: 92139427
< Connection: close
< Alt-Svc: hq=":443"; ma=2592000; quic=51303431; quic=51303339; quic=51303338; quic=51303337; quic=51303335,quic=":443"; ma=2592000; v="41,39,38,37,35"
< X-Content-Type-Options: nosniff
< Server: gvs 1.0
<
{ [5 bytes data]
* Closing connection 0
} [5 bytes data]
* TLSv1.2 (OUT), TLS alert, Client hello (1):
} [2 bytes data]
```
I might be wrong, but I can't see any illegal characters that might cause the URL parsing to fail.
```
$ printf %s "https://r1---sn-nu5gi0c-npok.googlevideo.com/videoplayback?id=7a33ded9e6218d1e&itag=137&source=youtube&requiressl=yes&ei=V0knWt3fBIbsowOg1IfgCg&mn=sn-nu5gi0c-npok&mm=31&ms=au&mv=u&pl=43&ratebypass=yes&mime=video/mp4&gir=yes&clen=92139427&lmt=1512416704858018&dur=195.153&signature=329F0B18B27DB4AA9F6F9C8BF58835F8984356C8.FBE391BCB4F0C2A7BEDF79D7635AAD71E92886&mt=1512523703&key=dg_yt0&ip=2406:3000:11:1022:9d80:1845:6e37:751d&ipbits=0&expire=1512545719&sparams=ip,ipbits,expire,id,itag,source,requiressl,ei,mn,mm,ms,mv,pl,ratebypass,mime,gir,clen,lmt,dur" | xxd
00000000: 6874 7470 733a 2f2f 7231 2d2d 2d73 6e2d https://r1---sn-
00000010: 6e75 3567 6930 632d 6e70 6f6b 2e67 6f6f nu5gi0c-npok.goo
00000020: 676c 6576 6964 656f 2e63 6f6d 2f76 6964 glevideo.com/vid
00000030: 656f 706c 6179 6261 636b 3f69 643d 3761 eoplayback?id=7a
00000040: 3333 6465 6439 6536 3231 3864 3165 2669 33ded9e6218d1e&i
00000050: 7461 673d 3133 3726 736f 7572 6365 3d79 tag=137&source=y
00000060: 6f75 7475 6265 2672 6571 7569 7265 7373 outube&requiress
00000070: 6c3d 7965 7326 6569 3d56 306b 6e57 7433 l=yes&ei=V0knWt3
00000080: 6642 4962 736f 774f 6731 4966 6743 6726 fBIbsowOg1IfgCg&
00000090: 6d6e 3d73 6e2d 6e75 3567 6930 632d 6e70 mn=sn-nu5gi0c-np
000000a0: 6f6b 266d 6d3d 3331 266d 733d 6175 266d ok&mm=31&ms=au&m
000000b0: 763d 7526 706c 3d34 3326 7261 7465 6279 v=u&pl=43&rateby
000000c0: 7061 7373 3d79 6573 266d 696d 653d 7669 pass=yes&mime=vi
000000d0: 6465 6f2f 6d70 3426 6769 723d 7965 7326 deo/mp4&gir=yes&
000000e0: 636c 656e 3d39 3231 3339 3432 3726 6c6d clen=92139427&lm
000000f0: 743d 3135 3132 3431 3637 3034 3835 3830 t=15124167048580
00000100: 3138 2664 7572 3d31 3935 2e31 3533 2673 18&dur=195.153&s
00000110: 6967 6e61 7475 7265 3d33 3239 4630 4231 ignature=329F0B1
00000120: 3842 3237 4442 3441 4139 4636 4639 4338 8B27DB4AA9F6F9C8
00000130: 4246 3538 3833 3546 3839 3834 3335 3643 BF58835F8984356C
00000140: 382e 4642 4533 3931 4243 4234 4630 4332 8.FBE391BCB4F0C2
00000150: 4137 4245 4446 3739 4437 3633 3541 4144 A7BEDF79D7635AAD
00000160: 3731 4539 3238 3836 266d 743d 3135 3132 71E92886&mt=1512
00000170: 3532 3337 3033 266b 6579 3d64 675f 7974 523703&key=dg_yt
00000180: 3026 6970 3d32 3430 363a 3330 3030 3a31 0&ip=2406:3000:1
00000190: 313a 3130 3232 3a39 6438 303a 3138 3435 1:1022:9d80:1845
000001a0: 3a36 6533 373a 3735 3164 2669 7062 6974 :6e37:751d&ipbit
000001b0: 733d 3026 6578 7069 7265 3d31 3531 3235 s=0&expire=15125
000001c0: 3435 3731 3926 7370 6172 616d 733d 6970 45719&sparams=ip
000001d0: 2c69 7062 6974 732c 6578 7069 7265 2c69 ,ipbits,expire,i
000001e0: 642c 6974 6167 2c73 6f75 7263 652c 7265 d,itag,source,re
000001f0: 7175 6972 6573 736c 2c65 692c 6d6e 2c6d quiressl,ei,mn,m
00000200: 6d2c 6d73 2c6d 762c 706c 2c72 6174 6562 m,ms,mv,pl,rateb
00000210: 7970 6173 732c 6d69 6d65 2c67 6972 2c63 ypass,mime,gir,c
00000220: 6c65 6e2c 6c6d 742c 6475 72 len,lmt,dur
``` | cant-reproduce | low | Critical |
279,625,016 | go | all: announce end of support for old macOS releases | **Note, Aug 2022: See https://github.com/golang/go/issues/23011#issuecomment-738395341 for the macOS deprecation schedule.**
- - -
Apple continues to put out new macOS releases. We can't run builders for all of them forever.
I propose we announce in the Go 1.10 release notes that Go 1.10 will be the last release to officially support macOS 10.8 (Mountain Lion).
macOS 10.8 was last updated Oct 3, 2013, over 4 years ago.
(macOS 10.9 was last updated Mar 21, 2016, 19 months ago, which is somewhat more recent)
Apple doesn't publish official End-of-Life dates for macOS versions, but I read that their security policy is that they issue security updates for the past 3 releases.
Given that they're on 10.13 now, that means 10.13, 10.12, and 10.11 are supported by them.
Our policy of additionally supporting 10.10 and 10.9 in Go 1.11 would be even more.
/cc @rsc @ianlancetaylor | Documentation,Builders,NeedsFix,release-blocker,recurring | high | Critical |
279,712,900 | react-native | Image component with local uri source (file://) does not render initially | Image components with local uri sources (file://) do not render initially. When a re-render of the component tree happens, the images get rendered.
### Environment
Environment:
OS: macOS High Sierra 10.13.1
Node: 8.9.1
Yarn: 1.3.2
npm: 5.5.1
Watchman: 4.9.0
Xcode: 9.1 (9B55)
Android Studio: 3.0.1
Packages: (wanted => installed)
react-native: 0.51.0 (@sraka1 reports this is still an issue in 0.58.4)
react: 16.0.0
Target Platform: Android (5.0.0)
### Steps to Reproduce
```javascript
const image = {uri: 'http://my.bamps.online/cfs/files/images/D8Xg27puAiqjvKetD?store=normal'}
// image2 is cached version of image by react-native-cached-image
const image2 = {uri: 'file:///data/data/com.testcachedimage/cache/imagesCacheDir/my_bamps_online_e6d51c26611343fb5e8c3e493674423c3d3f3943/38baef384cbb5bcc5f6138ca0653884561f85fde.jpg'}
export default class App extends Component<{}> {
render() {
return (
<View style={{backgroundColor:'#777'}}>
<Image source={image2} style={{width: 100, height: 100}}/>
<Image source={image2} style={{width: 300, height: 100}}/>
<ImageBackground source={image2} style={{width: 100, height: 100}}/>
<ImageBackground source={image2} style={{width: 300, height: 100}}/>
<Image source={image} style={{width: 100, height: 100}}/>
<Image source={image} style={{width: 300, height: 100}}/>
</View>
);
}
}
```
### Actual Behavior
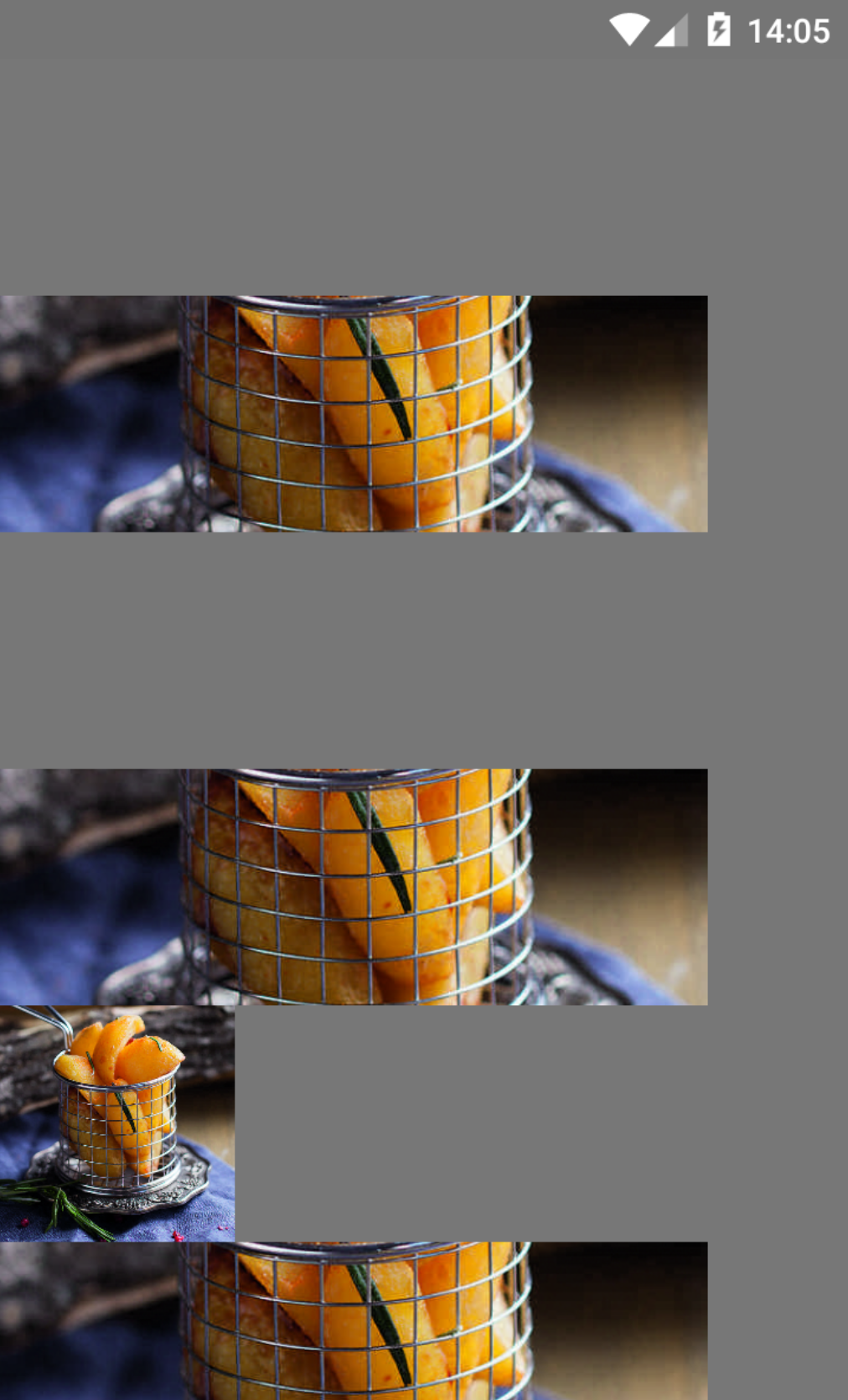
| Help Wanted :octocat:,Ran Commands,Platform: Android,Component: Image,Bug | medium | Critical |
279,715,373 | angular | View encapsulation is emulated when importing a file inside `::ng-deep` | <!--
PLEASE HELP US PROCESS GITHUB ISSUES FASTER BY PROVIDING THE FOLLOWING INFORMATION.
ISSUES MISSING IMPORTANT INFORMATION MAY BE CLOSED WITHOUT INVESTIGATION.
-->
## I'm submitting a...
<!-- Check one of the following options with "x" -->
<pre><code>
[ ] Regression (a behavior that used to work and stopped working in a new release)
[x] Bug report <!-- Please search GitHub for a similar issue or PR before submitting -->
[ ] Feature request
[ ] Documentation issue or request
[ ] Support request => Please do not submit support request here, instead see https://github.com/angular/angular/blob/master/CONTRIBUTING.md#question
</code></pre>
## Current behavior
<!-- Describe how the issue manifests. -->
The following .scss file with the default `Emulated` view encapsulation...
```
::ng-deep {
@import '~some/file.css`
}
```
...results in `[_ngcontent-${id}]` attributes applied to its content.
## Expected behavior
<!-- Describe what the desired behavior would be. -->
They should not be added because it's inside `::ng-deep`.
## Minimal reproduction of the problem with instructions
<!--
For bug reports please provide the *STEPS TO REPRODUCE* and if possible a *MINIMAL DEMO* of the problem via
https://plnkr.co or similar (you can use this template as a starting point: http://plnkr.co/edit/tpl:AvJOMERrnz94ekVua0u5).
-->
I think the example above is pretty straightforward, but I can create a repro repo if requested.
## What is the motivation / use case for changing the behavior?
<!-- Describe the motivation or the concrete use case. -->
Being able to include a third-party css file without view encapsulation being applied to it.
## Environment
<pre><code>
Angular version: 5.0.0
<!-- Check whether this is still an issue in the most recent Angular version -->
| type: bug/fix,freq1: low,area: core,core: CSS encapsulation,P5 | low | Critical |
279,727,704 | TypeScript | CFA should consider branch flags | This is a suggestion to improve the CFA. It would be good if TypeScript considered branch flags when analysing code paths.
```ts
function f() {
let text: string;
let hasTakenVerySpecificBranch = false;
if (0.5 < Math.random()) {
if (0.5 < Math.random()) {
if (0.5 < Math.random()) {
hasTakenVerySpecificBranch = true;
text = '';
}
}
}
if (!hasTakenVerySpecificBranch) {
text = ''
}
console.log(text); // Error 'text' is used before assigned.
}
```
We assign `text` on all possible code paths above. And it shouldn't error above.
A branch flag is simply a boolean variable that is considered when doing the control flow analysis. | Suggestion,Needs Proposal | low | Critical |
279,733,620 | angular | [Animations, Angular 5] inner element is not animated | <!--
PLEASE HELP US PROCESS GITHUB ISSUES FASTER BY PROVIDING THE FOLLOWING INFORMATION.
ISSUES MISSING IMPORTANT INFORMATION MAY BE CLOSED WITHOUT INVESTIGATION.
-->
## I'm submitting a...
<!-- Check one of the following options with "x" -->
<pre><code>
[x] Regression (a behavior that used to work and stopped working in a new release)
[ ] Bug report <!-- Please search GitHub for a similar issue or PR before submitting -->
[ ] Feature request
[ ] Documentation issue or request
[ ] Support request => Please do not submit support request here, instead see https://github.com/angular/angular/blob/master/CONTRIBUTING.md#question
</code></pre>
## Current behavior
<!-- Describe how the issue manifests. -->
When the animation is used for the parent element, the animation for the child element does not work.
## Expected behavior
<!-- Describe what the desired behavior would be. -->
I expect that the animation for the inner element will work at the same time with the animation of the parent, since it worked before.
## Minimal reproduction of the problem with instructions
<!--
For bug reports please provide the *STEPS TO REPRODUCE* and if possible a *MINIMAL DEMO* of the problem via
https://plnkr.co or similar (you can use this template as a starting point: http://plnkr.co/edit/tpl:AvJOMERrnz94ekVua0u5).
-->
http://embed.plnkr.co/9JUga9LYw0NEDmdSempP/
it works as expected if set angular version ^4.2.0
(Also works as expected with the version set to 5.0.2, but breaks in 5.0.3. thx **jamesthurley**)
## What is the motivation / use case for changing the behavior?
<!-- Describe the motivation or the concrete use case. -->
To animate nested elements.
## Environment
<pre><code>
Angular version: 5.0.5
<!-- Check whether this is still an issue in the most recent Angular version -->
Browser:
- [x] Chrome (desktop) version 62
For Tooling issues:
- Node version: 8.9.1 <!-- run `node --version` -->
- Platform: Win 10 <!-- Mac, Linux, Windows -->
Others:
<!-- Anything else relevant? Operating system version, IDE, package manager, HTTP server, ... -->
</code></pre> | type: bug/fix,area: animations,freq3: high,P4 | medium | Critical |
279,851,613 | angular | Animation on query :leave does not apply on lazy-loaded children components | <!--
PLEASE HELP US PROCESS GITHUB ISSUES FASTER BY PROVIDING THE FOLLOWING INFORMATION.
ISSUES MISSING IMPORTANT INFORMATION MAY BE CLOSED WITHOUT INVESTIGATION.
-->
## I'm submitting a...
<!-- Check one of the following options with "x" -->
<pre><code>
[ ] Regression (a behavior that used to work and stopped working in a new release)
[x] Bug report <!-- Please search GitHub for a similar issue or PR before submitting -->
[ ] Feature request
[ ] Documentation issue or request
[ ] Support request => Please do not submit support request here, instead see https://github.com/angular/angular/blob/master/CONTRIBUTING.md#question
</code></pre>
## Current behavior
<!-- Describe how the issue manifests. -->
query(':leave' animation has no effect on lazy loaded child components. The component simply disappears
## Expected behavior
<!-- Describe what the desired behavior would be. -->
The leave query should take effect on lazy loaded child components. I appears that it was affected by changes made in 5.0.4
In 5.0.3 animation is happening, however with some sort of a slight delay
## Minimal reproduction of the problem with instructions
<!--
For bug reports please provide the *STEPS TO REPRODUCE* and if possible a *MINIMAL DEMO* of the problem via
https://plnkr.co or similar (you can use this template as a starting point: http://plnkr.co/edit/tpl:AvJOMERrnz94ekVua0u5).
-->
https://embed.plnkr.co/Nfj70L0RzFnAT8e4eC2I/
Animation on routing is in place. Notice that the lazyloaded components animation is not triggering onLeave
## What is the motivation / use case for changing the behavior?
<!-- Describe the motivation or the concrete use case. -->
## Environment
5.0.4
<pre><code>
Angular version: X.Y.Z
<!-- Check whether this is still an issue in the most recent Angular version -->
Browser:
- [x] Chrome (desktop) version XX
- [ ] Chrome (Android) version XX
- [ ] Chrome (iOS) version XX
- [ ] Firefox version XX
- [ ] Safari (desktop) version XX
- [ ] Safari (iOS) version XX
- [ ] IE version XX
- [ ] Edge version XX
For Tooling issues:
- Node version: XX <!-- run `node --version` -->
- Platform: <!-- Mac, Linux, Windows -->
Others:
<!-- Anything else relevant? Operating system version, IDE, package manager, HTTP server, ... -->
</code></pre>
| type: bug/fix,area: animations,freq2: medium,P3 | low | Critical |
280,177,152 | rust | Confusing error message with mismatched `proc-macro-derive` dependencies | Not sure if this is that urgent, but it took me several hours to debug this simple compiler error with help from multiple people, so raising anyway...
Suppose you have a crate `foo` defining some trait `Foo`, and then a `foo-derive` crate of type `proc-macro-lib` that allows you to custom derive `Foo`. `foo-derive` must then depend on `foo` in `Cargo.toml`, because it mentions the `Foo` trait. If I then want to use the derivation I have to use *both* `foo` and `foo-derive` crates. Fine.
The problem comes if the using crate (let's call it `bar`) uses a different version of `foo` from the one that `foo-derive` does. So let's say that `foo-derive` 1.0 has in `Cargo.toml`:
```toml
[dependencies]
foo = "1.1"
```
Now, if in `bar`'s `Cargo.toml` I mistakenly add:
```toml
[dependencies]
foo = "1.0"
foo-derive = "1.0"
```
And then use the custom derive of `Foo` within `bar`:
```rust
`#[derive(foo)]`
struct BarStruct{ ... }
```
I get the confusing error `the trait Foo is not implemented for BarStruct`. Even though I can see using cargo-expand that the trait *is* implemented. The point is that the derive code has implemented a *different version* of `Foo`, and the fix is to change `bar` to depend on `foo` version 1.1. But the compiler error does not make that at all obvious! | A-diagnostics,T-compiler,A-proc-macros,D-crate-version-mismatch | low | Critical |
280,186,840 | pytorch | Feature request: Correlation module | This module was used by FlowNetC, it is described [here](https://arxiv.org/pdf/1504.06852.pdf) (in section 3.)
Here is the caffe implementation of the authors
https://github.com/lmb-freiburg/flownet2/blob/master/src/caffe/layers/correlation_layer.cu
Here is one tensorflow implementation
https://github.com/sampepose/flownet2-tf/tree/master/src/ops/correlation
And also NVIDIA recently released a custom pytorch operator here
https://github.com/NVIDIA/flownet2-pytorch/tree/master/networks/correlation_package
here is another attempt in pytorch (but only high level)
https://github.com/onlytailei/pytorch-flownet2/blob/master/nets/flownetC.py#L45
Would it be useful to add such a feature in functional pytorch library ? | triaged | medium | Critical |
280,228,418 | rust | Result from format_args! cannot be center-aligned within another format string | I can do `println!("{:=^80}", " TEST ")` to pretty-print a center-aligned header within equality signs.
However, the alignment is ignored when I do `println!("{:=^80}", format_args!(" TEST "))`.
I need the latter syntax to further write code like
`println!("{:=^80}", format_args!(" TEST {:#016X} ", some_integer))`.
I understand that a `format_args!` result is harder to center-align due to cases where the final width could be unknown. Nevertheless, it should be possible for the compiler to determine the string width in my example cases to let it be center-aligned. | C-enhancement,A-macros,T-libs-api | low | Major |
280,252,805 | vscode | Feature Request: Keybinding Overloading | <!-- Do you have a question? Please ask it on http://stackoverflow.com/questions/tagged/vscode. -->
I'm *not* asking for the ability to run multiple commands *in sequence*, as mentioned in
* https://github.com/Microsoft/vscode/issues/18621
* https://github.com/Microsoft/vscode/issues/871
* https://github.com/Microsoft/vscode/issues/171
or as addressed with the [macros extension](https://marketplace.visualstudio.com/items?itemName=geddski.macros)
I'd like to be able to assign a given keybinding to multiple different commands ***separately***, and if multiple commands are applicable in the current context when the keystroke is used, present the user with a menu of the options, *rather than considering it a conflict*, where only one can "win".
There are *many* ***significant*** benefits to this:
* You don't have to worry about keybindings from your multiple extensions conflicting with each other.
* All extension authors can add the keybinding they think makes the most sense, instead of telling you to manually add it in your own personal keybindings.json.
* You don't have to worry about your personal keybindings clobbering a default keybinding (this sort of conflict does not appear when you use "Show Conflicts"—at least it did not in my tests).
* You will never accidentally use the wrong command because of a conflict in which you expected another command to be triggered by your keybinding.
* You can purposefully add multiple related commands to the same keybinding, so that you have fewer bindings to memorize. For example,
+ you could assign all of your Git-related commands to <kbd>⌘</kbd><kbd>⌥</kbd><kbd>G</kbd>, or, for more focused groupings,
+ you could assign all variations of Git commands related to adding files to <kbd>⌘</kbd><kbd>⌥</kbd><kbd>A</kbd>, and those related to commiting to <kbd>⌘</kbd><kbd>⌥</kbd><kbd>C</kbd>, and so forth.
* You can experiment with changed keybindings without having to change the whole world in order to accommodate the change you think you might prefer.
Since there may be people who have grown accustomed to existing conflicts in their keybindings (and accustomed to the current winner) it would make sense for this behavior to have a setting to turn it on or off, so that they are not presented with a menu containing what used to be considered "conflicts" but under the new behavior are simply alternate options assigned to the same keystroke. | feature-request,keybindings | medium | Major |
280,288,071 | angular | JIT Complier needed with AOT Build for Dynamic Component. | <!--
PLEASE HELP US PROCESS GITHUB ISSUES FASTER BY PROVIDING THE FOLLOWING INFORMATION.
ISSUES MISSING IMPORTANT INFORMATION MAY BE CLOSED WITHOUT INVESTIGATION.
-->
## I'm submitting a...
<!-- Check one of the following options with "x" -->
<pre><code>
[ ] Regression (a behavior that used to work and stopped working in a new release)
[ ] Bug report <!-- Please search GitHub for a similar issue or PR before submitting -->
[X ] Feature request
[ ] Documentation issue or request
[ ] Support request => Please do not submit support request here, instead see https://github.com/angular/angular/blob/master/CONTRIBUTING.md#question
</code></pre>
## Current behavior
I have HTML templates which is loaded from database. It has Form Controls, which I need dynamically render.
Currently in Angular 4.0 it is working fine, but we are not able to update to Angular 5.0 since the feature is not available
## Expected behavior
I like to have the JIT Complier supported with AOT build. I am open to other option of displaying the controls.
## Minimal reproduction of the problem with instructions
For bug reports please provide the *STEPS TO REPRODUCE* and if possible a *MINIMAL DEMO* of the problem via
This is working example with uses the compiler to build.
The top template has the First Name and Last Name.
<div #container></div>
This container is loaded dynamically with Controls Address, City, Radio options.
http://plnkr.co/edit/rELaWPJ2cDJyCB55deTF?p=preview
For this to work in AOT build I change the Complier to JIT Complier
## What is the motivation / use case for changing the behavior?
All our use case are dynamically generated and it comes from Database. All validation are dynamically configured. Love to have the feature back.
## Environment
<pre><code>
Angular version: Working in Version 4.0.0, not working in Angular 5.0.0
<!-- Check whether this is still an issue in the most recent Angular version -->
Browser:
- [X ] Chrome (desktop) version XX
- [ ] Chrome (Android) version XX
- [ ] Chrome (iOS) version XX
- [ ] Firefox version XX
- [ ] Safari (desktop) version XX
- [ ] Safari (iOS) version XX
- [ ] IE version XX
- [ ] Edge version XX
For Tooling issues:
- Node version: XX <!-- run `node --version` -->
- Platform: <!-- Mac, Linux, Windows -->
Others:
<!-- Anything else relevant? Operating system version, IDE, package manager, HTTP server, ... -->
</code></pre>
| feature,area: core,area: compiler,feature: under consideration,compiler: jit | high | Critical |
280,295,918 | react | Consider removing XML compatibility from SSR or hiding it behind an option | See https://github.com/facebook/react/pull/11708#issuecomment-349953542.
Not sure if it's important but seems suboptimal to send extra markup if most people don't need XML. | Component: Server Rendering,Type: Breaking Change,React Core Team | low | Minor |
280,297,940 | go | go/ast: CommentMap heuristic sensitive to parentheses around unnamed result type | **Background**
I want to use structured comments to associate metadata with function parameter types in Go source code, with the function bodies automatically generated by an external tool.
**Problem**
There is a bad interaction between `(*printer.Config).Fprint` and `ast.NewCommentMap` for comments on function results.
If a function returns only one result, has an associated trailing comment, and lacks a body (e.g., because it is implemented in assembly), `NewCommentMap` will only associate the comment with the result if both are enclosed in parentheses. (Otherwise, the algorithm of `NewCommentMap` instead associates the comment with the entire file.)
Unfortunately, `(*printer.Config).Fprint` omits those parentheses, so running `gofmt` on such a file breaks the association between the metadata and the function result.
Example: https://play.golang.org/p/ovMOY6e5pB
```go
package foo
func Foo() (error /*metadata*/)
```
formats to
```go
package foo
func Foo() error /*metadata*/
```
**Proposal**
The problematic check is here:
https://github.com/golang/go/blob/7c46b62d0a6e2353db68da963c390b094e359a92/src/go/printer/nodes.go#L344-L348
I suspect it would be fixed by weakening that condition to:
```go
if n == 1 && result.List[0].Names == nil &&
(!result.Closing.IsValid() || !p.commentBefore(p.posFor(result.Closing)) {
```
| NeedsFix | low | Critical |
280,310,679 | angular | Cannot test JSONP HTTP request using the HttpClientTestingModule and HttpTestingController | <!--
PLEASE HELP US PROCESS GITHUB ISSUES FASTER BY PROVIDING THE FOLLOWING INFORMATION.
ISSUES MISSING IMPORTANT INFORMATION MAY BE CLOSED WITHOUT INVESTIGATION.
-->
## I'm submitting a...
<!-- Check one of the following options with "x" -->
<pre><code>
[ ] Regression (a behavior that used to work and stopped working in a new release)
[x] Bug report <!-- Please search GitHub for a similar issue or PR before submitting -->
[ ] Feature request
[ ] Documentation issue or request
[ ] Support request => Please do not submit support request here, instead see https://github.com/angular/angular/blob/master/CONTRIBUTING.md#question
</code></pre>
## Current behavior
<!-- Describe how the issue manifests. -->
When I try to mock a JSONP HTTP request using the HttpClientTestingModule, I get the error
`Error: Expected one matching request for criteria "Match URL: https://archive.org/index.php?output=json&callback=callback", found none.`
I posted a [stackoverflow question](https://stackoverflow.com/questions/47703877/how-do-i-test-a-jsonp-http-request-in-angular) about this issue.
## Expected behavior
<!-- Describe what the desired behavior would be. -->
A TestRequest to be returned from `httpMock.expectOne(service.url);` in the following code sample.
## Minimal reproduction of the problem with instructions
<!--
For bug reports please provide the *STEPS TO REPRODUCE* and if possible a *MINIMAL DEMO* of the problem via
https://plnkr.co or similar (you can use this template as a starting point: http://plnkr.co/edit/tpl:AvJOMERrnz94ekVua0u5).
-->
I'm using the [HttpClientModule][1] and [HttpClientJsonpModule][2] to make a JSONP HTTP request in a service.
**app.module.ts**
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { HttpClientModule, HttpClientJsonpModule } from '@angular/common/http';
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
HttpClientModule,
HttpClientJsonpModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
This service uses the [jsonp][3] method from the [HttpClient class][4] to get a JSONP response from the specified URL.
**example.service.ts**
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
@Injectable()
export class ExampleService {
url = "https://archive.org/index.php?output=json&callback=callback";
constructor(private http: HttpClient) { }
getData() {
return this.http.jsonp(this.url, 'callback');
}
}
Using the [HttpClientTestingModule][7], I inject the [HttpTestingController][8] so I can mock and flush my JSONP HTTP request.
**example.service.spec.ts**
import { TestBed, inject } from '@angular/core/testing';
import {
HttpClientTestingModule,
HttpTestingController
} from '@angular/common/http/testing';
import { ExampleService } from './example.service';
describe('ExampleService', () => {
let service: ExampleService;
let httpMock: HttpTestingController;
beforeEach(() => {
TestBed.configureTestingModule({
imports: [HttpClientTestingModule],
providers: [ExampleService]
});
service = TestBed.get(ExampleService);
httpMock = TestBed.get(HttpTestingController);
});
describe('#getData', () => {
it('should return an Observable<any>', () => {
const dummyData = { id: 1 };
service.getData().subscribe(data => {
expect(data).toEqual(dummyData);
});
const req = httpMock.expectOne(service.url); // Error
expect(req.request.method).toBe('JSONP');
req.flush(dummyData);
});
});
});
If I change the request method to GET, this test works as expected.
Also, on Gitter @alxhub showed me that I could get the expected return value from `httpMock.expectOne(service.url);` if I change `example.service.spec.ts` to the following:
import { TestBed, inject } from '@angular/core/testing';
import {
HttpClientJsonpModule,
HttpBackend,
JsonpClientBackend
} from '@angular/common/http';
import {
HttpClientTestingModule,
HttpTestingController
} from '@angular/common/http/testing';
import { ExampleService } from './example.service';
describe('ExampleService', () => {
let service: ExampleService;
let httpMock: HttpTestingController;
beforeEach(() => {
TestBed.configureTestingModule({
imports: [HttpClientTestingModule],
providers: [ExampleService, { provide: JsonpClientBackend, useExisting: HttpBackend }]
});
service = TestBed.get(ExampleService);
httpMock = TestBed.get(HttpTestingController);
});
describe('#getData', () => {
it('should return an Observable<any>', () => {
const dummyData = { id: 1 };
service.getData().subscribe(data => {
expect(data).toEqual(dummyData);
});
const req = httpMock.expectOne(request => request.url === service.url);
expect(req.request.method).toBe('JSONP');
req.flush(dummyData);
});
});
});
[1]: https://angular.io/api/common/http/HttpClientModule
[2]: https://angular.io/api/common/http/HttpClientJsonpModule
[3]: https://angular.io/api/common/http/HttpClient#jsonp
[4]: https://angular.io/api/common/http/HttpClient
[5]: https://angular.io/api/common/http/JsonpInterceptor
[6]: https://angular.io/api/common/http/JsonpClientBackend
[7]: https://angular.io/api/common/http/testing/HttpClientTestingModule
[8]: https://angular.io/api/common/http/testing/HttpTestingController
## What is the motivation / use case for changing the behavior?
<!-- Describe the motivation or the concrete use case. -->
To be able to test JSONP HTTP requests using the HttpClientTestingModule and HttpTestingController.
## Environment
<pre><code>
Angular version: 5.0.5
<!-- Check whether this is still an issue in the most recent Angular version -->
Browser:
- [x] Chrome (desktop) version XX
- [ ] Chrome (Android) version XX
- [ ] Chrome (iOS) version XX
- [ ] Firefox version XX
- [ ] Safari (desktop) version XX
- [ ] Safari (iOS) version XX
- [ ] IE version XX
- [ ] Edge version XX
For Tooling issues:
- Node version: 8.9.1 <!-- run `node --version` -->
- Platform: Mac OS 10.13.1 <!-- Mac, Linux, Windows -->
Others:
<!-- Anything else relevant? Operating system version, IDE, package manager, HTTP server, ... -->
</code></pre>
| type: bug/fix,freq1: low,area: common/http,state: confirmed,design complexity: low-hanging,P4 | low | Critical |
280,316,720 | TypeScript | In JSDocs notation for index signatures, Object and object should be treated as the same | <!-- BUGS: Please use this template. -->
<!-- Please try to reproduce the issue with `typescript@next`. It may have already been fixed. -->
**TypeScript Version:** 2.7.0-dev.201xxxxx
**Code**
```ts
/**
* Test.
*
* @param {object.<string, number>} obj An object.
*/
function func(obj) {
console.log(obj);
}
```
**Expected behavior:**
`obj` should have a index signature as in #15105
**Actual behavior:**
`obj` is treated as `any`
**Additional Info:**
This was mentioned in the above issue, and it seems to be related to the way Typescript manage `Object` and `object`, but in JSDocs, they are treated similar. | Suggestion,In Discussion,Domain: JSDoc | low | Critical |
280,325,856 | TypeScript | Takes a while with high memory to calculate highly recursive types with intersections | <!-- BUGS: Please use this template. -->
<!-- QUESTIONS: This is not a general support forum! Ask Qs at http://stackoverflow.com/questions/tagged/typescript -->
<!-- SUGGESTIONS: See https://github.com/Microsoft/TypeScript-wiki/blob/master/Writing-Good-Design-Proposals.md -->
<!-- Please try to reproduce the issue with `typescript@next`. It may have already been fixed. -->
**TypeScript Version:** 2.6.2
**Code**
Apologies for the length - I can't really make the repro any smaller than this without it taking a fraction of the time and about half the memory. Also, note: this does not require a config.
```ts
interface Context<P, O> {
createV(this: this & Context<P, O & {raw: true}>, attrs: P): Context<P, O>;
createRC(this: this & Context<P, O & {raw: true}>, attrs: P, ref: (elem: P) => any): Context<P, O>;
simpleV(this: this & Context<P, O & {raw: true}>, attrs: P): void;
simpleRC(this: this & Context<P, O & {raw: true}>, attrs: P, ref: (elem: P) => any): void;
add(this: this & Context<P, O & {text: true}>): void;
raw(this: this & Context<P, O & {raw: true}>, elem: P): void;
invokeC<C>(
tag: Component<object, P, O>,
children: (r: Context<P, O>, context: C) => void,
context: C
): void;
invokeAC<A extends object, C>(
tag: Component<A, P, O>, attrs: A,
children: (r: Context<P, O>, context: C) => void,
context: C
): void;
}
interface TopContext<P, O> extends Context<P, O> {
hasOption<K extends keyof O>(key: K): this is (this & TopContext<P, {[P in K]: O[P]}>);
}
type Component<A extends object, P, O> = <C>(
attrs: A, r: TopContext<P, O>,
children: (r: Context<P, O>, context: C) => void,
context: C
) => void
interface ContextWrap<P, O> {
instance: Context<P, O>;
}
interface TopContextWrap<P, O> extends ContextWrap<P, O> {
instance: TopContext<P, O>;
}
```
**Expected behavior:** It to take maybe a few seconds. Remove any one function or property out of that, and that's the compilation time I would normally expect.
**Actual behavior:** It takes ~15-20 seconds to check, and requires ~180-200 MB of memory in the process.
| Bug | low | Critical |
280,325,956 | go | x/build: unify gomote push and gomote ssh users | When I "gomote push" to a gomote host, it writes all of the files and directories it copies over as user "root".
When I "gomote ssh", it logs me in as "gopher". At that point, I don't have permissions to modify any of the files or directories, so things like all.bash don't work when it tries to write anything.
```
$ ./all.bash
Building Go cmd/dist using /tmp/workdir/go1.4.
go install _/tmp/workdir/go/src/cmd/dist: open cmd/dist/dist: permission denied
```
At least, this is true on openbsd-amd64.
It would also be nice to set GOROOT_BOOTSTRAP to /tmp/workdir/go1.4 when ssh-ing in.
But only if it was already gomote pushed?
@bradfitz | Builders,NeedsFix | low | Minor |
280,331,700 | TypeScript | Completions are low-quality for UMD modules without typings | For example, reference `oidc-client.js` from the corresponding [npm package](https://www.npmjs.com/package/oidc-client), instantiate an `Oidc.UserManager`, and dot into the resulting object.
Expected: properties of `Oidc.UserManager` are offered
Actual: identifiers appearing in file are offered (i.e. min-bar experience). | Suggestion,Needs Proposal,Domain: JavaScript | low | Minor |
280,364,247 | rust | Spurious confusing errors on trait impl on type name collision | ```rust
type Type = ();
#[derive(Debug)]
struct Type;
impl Iterator for Type {}
```
Gives:
```
Compiling playground v0.0.1 (file:///playground)
error[E0428]: the name `Type` is defined multiple times
--> src/main.rs:4:1
|
1 | type Type = ();
| --------------- previous definition of the type `Type` here
...
4 | struct Type;
| ^^^^^^^^^^^^ `Type` redefined here
|
= note: `Type` must be defined only once in the type namespace of this module
error[E0119]: conflicting implementations of trait `std::fmt::Debug` for type `()`:
--> src/main.rs:3:10
|
3 | #[derive(Debug)]
| ^^^^^
|
= note: conflicting implementation in crate `core`
error[E0117]: only traits defined in the current crate can be implemented for arbitrary types
--> src/main.rs:6:1
|
6 | impl Iterator for Type {}
| ^^^^^^^^^^^^^^^^^^^^^^^^^ impl doesn't use types inside crate
|
= note: the impl does not reference any types defined in this crate
= note: define and implement a trait or new type instead
error[E0117]: only traits defined in the current crate can be implemented for arbitrary types
--> src/main.rs:3:10
|
3 | #[derive(Debug)]
| ^^^^^ impl doesn't use types inside crate
|
= note: the impl does not reference any types defined in this crate
= note: define and implement a trait or new type instead
error: aborting due to 4 previous errors
error: Could not compile `playground`.
To learn more, run the command again with --verbose.
```
The first error is true. The rest are spurious. There are probably other spurious errors that can be generated via name collision. | C-enhancement,A-diagnostics,T-compiler | low | Critical |
280,369,794 | godot | Messed up animation when importing 3D scene DAE | **Operating system or device, Godot version, GPU Model and driver (if graphics related):**
godot 3 (tip), ubuntu
**Issue description:**
When importing a DAE that contains a rigged mesh and animation exported from blender using the better collada exporter, the resulting animation flips around and generally misbehaves.
Here's an example video, blender is running on the right (slowly) and the equivalent import for godot is on the left.
https://www.youtube.com/watch?v=fBVpZ2v6Nt4
EDIT: updated link
| bug,topic:import,topic:animation,topic:3d | medium | Major |
280,422,935 | youtube-dl | [youku] 1080P formats missing | C:\Users\lin>youtube-dl -F http://v.youku.com/v_show/id_XMzIwMzg4NTkyOA==.html?spm=a2hww.20027244.m_250379.5~1~3~A
[youku] XMzIwMzg4NTkyOA: Retrieving cna info
[youku] XMzIwMzg4NTkyOA: Downloading JSON metadata
ERROR: Youku server reported error -6004: 客户端无权播放,201; please report this issue on https://yt-dl.org/bug . Make sure you are using the latest version; type youtube-dl -U to update. Be sure to call youtube-dl with the --verbose flag and include its complete output.
C:\Users\lin>youtube-dl -U
youtube-dl is up-to-date (2017.12.02) | broken-IE | medium | Critical |
280,452,837 | pytorch | GridSampler behaviours | This is in continuity with #2625
Here are some observations with grid sample, that I think could be worth discussing :
### Normalized input
I figure that [-1, 1] bounding was enforced by original cuDNN API, but I cannot help noticing that most of the time, when not using grid generator, I have a pixel unit grid `[0, H-1]x[0, W-1]` which I have to normalize, and which is inverted back to pixel unit (see [here](https://github.com/pytorch/pytorch/blob/master/aten/src/THCUNN/SpatialGridSamplerBilinear.cu#L54) and [here](https://github.com/pytorch/pytorch/blob/master/aten/src/THNN/generic/SpatialGridSamplerBilinear.c#L71) ). Isn't it some kind of waste of computation ? maybe we can add an option to specify whether the input is normalized [-1, 1] or not [0, {H or W} -1]
### Optical Flow Sample
As discussed here NVIDIA/flownet2-pytorch#3 , resampling from an optical flow map is subotpimal. Assuming flow is normalized to fit nicely in a [-1, 1] normalized grid, You have to convert flow to grid. This can be done with affine grid generator, but you then have to generate an identity `theta` matrix, i.e. generate a `Bx3x2` zeros `theta` volatile Variable, fill `theta[:,0,0]` and `theta[:,1,1]` with ones, devolatilize the grid and add it to your normalized flow.
Along with dramatically simplyfying the workflow, would adding a `residual` option (or whatever its name could be) to implicitely add identity to the grid be a good solution ? (or maybe add a new `flow_sample` function ? )
### Border behaviour
This suggestion is admittedly only cosmetic as the resulting gradient would be the same as when 'same' padding is applied (from #3599), so obviously not essential. It could be nice to have zero padding, but no interpolation between 0s and actual values. See an example [here](https://discuss.pytorch.org/t/spatial-transformer-networks-boundary-grid-interpolation-behaviour/8891/2) . As soon as grid value is outside [-1,1], output 0 as if point was far outside the input (and thus no interpolation between void and the border)
This also could be beneficial for classifier associated with a pixel-wise loss for this particular resampled feature map. Classifier like 0 values for irrelevant data points, Pixel-wise loss likes non-zero gradients for valid values only
This is semantically different from 'same' padding so I assume it would not be easy just to add another mode to `padding_mode` option.
cc @csarofeen @ptrblck | module: cudnn,triaged | low | Minor |
280,573,163 | flutter | a11y: overlapping widgets don't block pointer events on iOS | Create an overlay over a dismissible barrier. The overlay should have same whitespace that doesn't allow user interaction between elements that do allow user interaction. During touch exploration, when the user now moves the finger into one of those whitespace areas, the a11y focus will move to the underlaying dismissible barrier. This can be very confusing and makes it hard to find the elements in the overlay that the user can interact with. | platform-ios,engine,a: accessibility,P3,team-ios,triaged-ios | low | Minor |
280,591,935 | TypeScript | Narrow the parent type of a singleton type to never in the false branch | If the type of the member checked is not falsy (and so is `never` in the false branch).
<!-- Please try to reproduce the issue with `typescript@next`. It may have already been fixed. -->
**TypeScript Version:** 2.7.0-dev.201xxxxx
**Code**
```ts
const x: {a: 1, b: 2} = { a: 1, b: 2 };
if (x.a) {
}
else {
x.a;
}
```
**Expected behavior:**
type of `x` in the `else` branch is `never`.
**Actual behavior:**
type of `x` is `{a: 1. b: 2}`, and `x.a` is (counterintuitively) `never`.
This would bring the behavior of truthiness guards in line with our new behavior for `in` type guards. | Suggestion,In Discussion | low | Minor |
280,596,038 | go | x/build: run DragonflyBSD VMs on GCE? | Looks like Dragonfly now supports virtio:
https://leaf.dragonflybsd.org/cgi/web-man?command=virtio§ion=4
So it should run on GCE?
If somebody could prepare make.bash scripts to script the install to prepare bootable images, we could run it on GCE.
See the netbsd, openbsd, and freebsd directories as examples: https://github.com/golang/build/tree/master/env
(The script must run on Linux and use qemu to do the image creation.)
/cc @tdfbsd
| help wanted,Builders,NeedsFix,new-builder,umbrella | high | Critical |
280,647,900 | flutter | When the build fails during flutter run, it says try --stacktrace or --info which don't work | ```
Run with --stacktrace option to get the stack trace. Run with --info or --debug option to get more log output.
Gradle build failed: 1
$ flutter run --stacktrace
Could not find an option named "stacktrace".
```
also --info doesn't work
| tool,t: gradle,a: first hour,a: quality,P2,team-tool,triaged-tool | low | Critical |
280,649,110 | go | x/net/html: incorrect handling nodes in the <head> section | Please answer these questions before submitting your issue. Thanks!
### What version of Go are you using (`go version`)?
go1.9.2 darwin/amd64
### Does this issue reproduce with the latest release?
Yes
### What operating system and processor architecture are you using (`go env`)?
GOARCH="amd64"
GOBIN=""
GOEXE=""
GOHOSTARCH="amd64"
GOHOSTOS="darwin"
GOOS="darwin"
### What did you do?
```
package main
import (
"bytes"
"fmt"
"golang.org/x/net/html"
"strings"
)
func main() {
s := "<html><head><meta/><a/><style></style></head><body></body></html>"
doc, _ := html.Parse(strings.NewReader(s))
var buf bytes.Buffer
if err := html.Render(&buf, doc); err == nil {
fmt.Println(buf.String())
}
}
```
### What did you expect to see?
Same structure as the input
```html
<html><head><meta/><a/><style></style></head><body></body></html>
```
### What did you see instead?
Nodes from <head> are moved into the <body> part
```html
<html><head><meta/></head><body><a><style></style></a></body></html>
```
| NeedsInvestigation | low | Major |
280,657,387 | flutter | TextSelection.isDirectional is not respected, make it do something useful eg: for Mac | It seems like it's not wired up anywhere. We should make it do something useful and test it or remove it.
It's current presence is confusing. | a: text input,framework,c: proposal,P2,team-text-input,triaged-text-input | low | Minor |
280,660,117 | go | cmd/compile: more helpful "is not a type" error when variable shadows type | ### What version of Go are you using (`go version`)?
Current playground version.
### Does this issue reproduce with the latest release?
Yes.
### What operating system and processor architecture are you using (`go env`)?
Unknown (plaground).
### What did you do?
The following code compiles with `fancyMap is not a type` errors in both `foo` and `foo2` ([playground](https://play.golang.org/p/Ms021WVI3f)):
```go
package main
type fancyMap = map[string]string
func foo(fancyMap fancyMap) {
var _ fancyMap
}
func foo2(fancyMap fancyMap) {
_ = make(fancyMap)
}
func main() {
}
```
### What did you expect to see?
Successful compilation or a more informative error message.
### What did you see instead?
Poor/incorrect error message. Simply renaming the `fancyMap` variable fixes the problem, but the error message is incorrect: `fancyMap` is a type.
Apologies if there's an existing issue for this.
| help wanted,NeedsFix | low | Critical |
280,729,200 | angular | Router • Allow to have a PreloadingStrategy in lazy loaded modules | <pre><code>
[ ] Regression
[ ] Bug report
[x] Feature request
[ ] Documentation issue or request
[ ] Support reques
</code></pre>
## Current behavior
Currently, we can only set a `PreloadingStrategy` from `forRoot` by using `config` like in `RouterModule.forRoot(routes: Routes, config?: ExtraOptions)`.
Why can't this be done in `forChild` `RouterModule.forChild(routes: Routes)`?
https://angular.io/api/router/RouterModule
## Expected behavior
Being able to preload a module from another lazy loaded module.
## What is the motivation / use case for changing the behavior?
My `registration` page is lazy loaded since only members subscribing need to load it.
The page right after could be lazy loaded. | feature,freq2: medium,area: router,router: lazy loading,feature: under consideration,feature: votes required | medium | Critical |
280,729,306 | kubernetes | client-go: keep API versions longer in the client library than in the apiserver | One pretty uniform feedback from client-go users @ KubeCon was that they struggle with supporting old and new Kubernetes clusters from one code-base of their operators. Keeping old API versions around longer than we serve them in kube-apiserver might help them. So they can move on to the new client-go, but have an abstraction logic in their operator to fall-back to the old API group versions.
Keeping API group versions around is cheap: we only have to keep them in k8s.io/api, but can remove the internal types and conversions. | priority/backlog,area/api,area/client-libraries,sig/api-machinery,kind/api-change,kind/feature,lifecycle/frozen | medium | Critical |
280,741,908 | TypeScript | Specifying lib: DOM and WebWorker should not be mutually exclusive | I got myself into a situation similar to #11093.
My project is designed to work either in the "main thread", with access to DOM, or as a WebWorker, where a different behavior kicks in. This was done for compatibility reasons and to have only one distributable js file and it's been working fine for the last two years.
In all this time I never specified the lib compiler option, only target ES5.
Today, as I'm experimenting some ES2015 stuff, I see that if I specify targets DOM,ES5,WebWorker,ES2015.Promise all hell breaks loose with errors. - Duplicate identifier, Subsequent variable declarations must have the same type.
Shouldn't this kind of mix be allowed in a project? | Suggestion,In Discussion | high | Critical |
280,741,962 | godot | Groups icons are visible in an instanciated scene | **Operating system or device, Godot version, GPU Model and driver (if graphics related):**
Windows 10, Godot beta1
**Issue description:**
I have a scene that is a CanvasLayer node with a few panels that have locked children nodes, this scene is within a player scene (KinematicBody2D) and that player scene is within a level scene (Node2D). the locations of the locked children nodes
 (this thing) move based on where they were in the inherited scene and not where they actually are on the canvas layer.
**Steps to reproduce:**
Make a CanvasLayer node, add some panels
Make a new scene on a kinematicbody2D, instance the canvaslayer
make a new scene, instance the kinematicbody2D, move it around on the editor, congrats.
**Link to minimal example project:**
CanvasLayer Scene
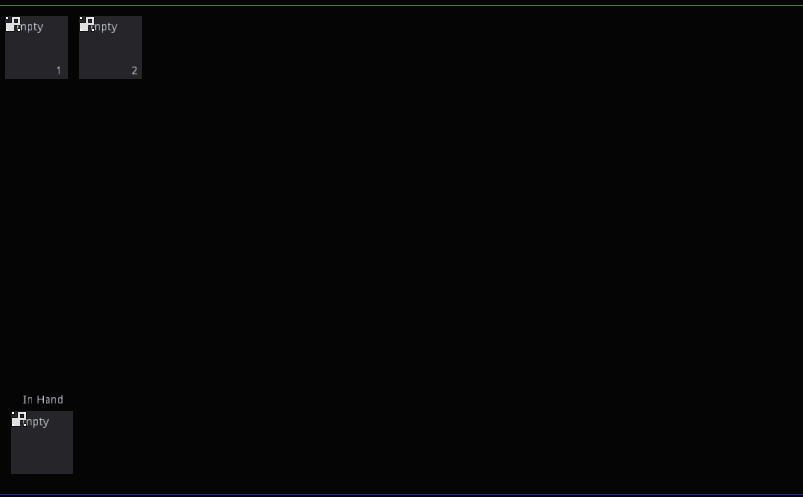
Player Scene
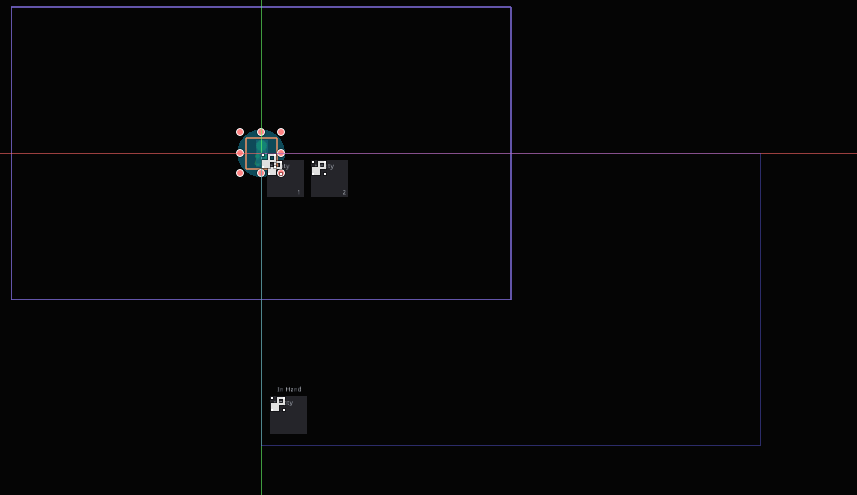
Level Scene
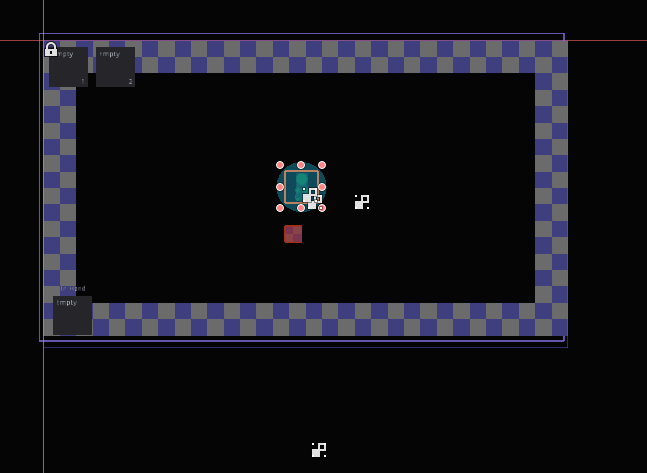
happy bug catching day. | bug,topic:editor | low | Critical |
280,742,109 | opencv | OpenCV 3.1.0 - Performance issue while focusing camera in Android. | OpenCV => 3.1.0
Operating System / Platform => Android
I installed apk for tutorial-1-camerapreview. It opens a camera, but it is taking more time to focus. And sometimes, it don't focus.
Whereas, when you open Camera using `new Intent(MediaStore.ACTION_IMAGE_CAPTURE)`, it focuses almost instantly.
Ofcourse, OpenCV performs some task in processing camera frame and that is causing lag in camera focusing, but it can be improved.
##### Steps to reproduce
1) Install APK for tutorial-1-camerapreview and observe time taken by camera to focus.
2) Open built-in camera App in you device and observe time taken by camera to focus.
Expected: The focusing of camera should be faster. | platform: android | low | Major |
280,745,321 | godot | Vertex Colored objects break ShaderMaterials | **Operating system or device, Godot version, GPU Model and driver (if graphics related):**
Tried on two different Windows 10 x64 PCs
**Issue description:**
In 3D, objects with Vertex Colors applied do not behave as expected with a shader applied. Objects appear to have no material.
**Steps to reproduce:**
Apply vertex color to object then export. Import into Godot.
Apply a shader material to the object. It will render correctly in the viewport. Upon running the game, the object will take the color of ambient light applied to it (Similar behavior to objects with no material)
**Link to minimal example project:**
Attached
[MaterialsTest.zip](https://github.com/godotengine/godot/files/1545030/MaterialsTest.zip)
| bug,platform:windows,topic:rendering | low | Major |
280,746,344 | rust | Backwards propagation of types leads to unhelpful errors [futures] | In general, and I genuinely wish I had a citation for this, developers likely tend to write their software going from input to output, even if the output is the eventual, fixed "goal" they would like to reach.
For example, the code here (git checkout available): https://git.neosmart.net/mqudsi/futuretest/src/rust-46606
```rust
let f = future::result(Ok(()))
.map_err(|()| "&'static str error")
.map(|_| future::result(Err("another &'static str error")))
.and_then(|_|
future::result(Ok(())
.map_err(|()| "String error".to_owned())
)
)
;
```
Two conflicting error types are mapped, the first is to `&'static str` and the second is to `String`, the [compiler output](https://git.neosmart.net/mqudsi/futuretest/src/rust-46606/cargo.out) indicates an error with the `&'static str` type instead of the `String` type usage:
```rust
Compiling futuretest v0.1.0 (file:///mnt/c/Users/Mahmoud/git/futuretest)
error[E0271]: type mismatch resolving `<futures::FutureResult<(), std::string::String> as futures::IntoFuture>::Error == &str`
--> src/main.rs:13:10
|
13 | .and_then(|_|
| ^^^^^^^^ expected struct `std::string::String`, found &str
|
= note: expected type `std::string::String`
found type `&str`
error[E0271]: type mismatch resolving `<futures::FutureResult<(), std::string::String> as futures::IntoFuture>::Error == &str`
--> src/main.rs:20:10
|
20 | core.run(f).unwrap();
| ^^^ expected struct `std::string::String`, found &str
|
= note: expected type `std::string::String`
found type `&str`
= note: required because of the requirements on the impl of `futures::Future` for `futures::AndThen<futures::Map<futures::MapErr<futures::FutureResult<(), ()>, [closure@src/main.rs:11:18: 11:43]>, [closure@src/main.rs:12:14: 12:67]>, futures::FutureResult<(), std::string::String>, [closure@src/main.rs:13:19: 16:14]>`
error: aborting due to 2 previous errors
error: Could not compile `futuretest`.
To learn more, run the command again with --verbose.
```
In an ideal situation, all types would match up and it wouldn't matter which line the type inference began with. But in the event of a type mismatch, such as here, the reported errors are based off the backwards type propagation, which doesn't seem right (opinion) and leads to an unhelpful error message (fact).
(To make the case a bit clearer, I included several uses of the correct type followed by a final usage of the incorrect type). | C-enhancement,A-diagnostics,T-compiler | low | Critical |
280,752,614 | rust | Move oom test out of line with -C panic=abort | The following code:
```rust
#[inline(never)]
pub fn foo() -> Vec<u8> {
vec![0; 900]
}
```
compiles to
```assembly
example::foo:
push rbp
mov rbp, rsp
push rbx
sub rsp, 40
mov rbx, rdi
lea rdx, [rbp - 32]
mov edi, 900
mov esi, 1
call __rust_alloc_zeroed@PLT
test rax, rax
je .LBB0_1
mov qword ptr [rbx], rax
mov qword ptr [rbx + 8], 900
mov qword ptr [rbx + 16], 900
mov rax, rbx
add rsp, 40
pop rbx
pop rbp
ret
.LBB0_1:
mov rax, qword ptr [rbp - 32]
movups xmm0, xmmword ptr [rbp - 24]
movaps xmmword ptr [rbp - 48], xmm0
mov qword ptr [rbp - 32], rax
movaps xmm0, xmmword ptr [rbp - 48]
movups xmmword ptr [rbp - 24], xmm0
lea rdi, [rbp - 32]
call __rust_oom@PLT
ud2
```
It would be a good code size improvement for the oom handling to be moved into __rust_alloc_zeroed when compiling with panic=abort. This will also make the branch on null better predicted because it's not duplicated all around the code base, but in just one spot. | I-slow,C-enhancement,A-allocators,T-compiler | low | Major |
280,778,773 | rust | Confusing error message when trait is impl'd on &T and bounds are not satisfied | Example code: https://play.rust-lang.org/?gist=6e7c1a4ad58c82717271ad4d5cef1181&version=stable
The form that I encountered was:
```
error[E0277]: the trait bound `&Ident: std::cmp::PartialEq<str>` is not satisfied
--> src/main.rs:22:18
|
22 | if ident.eq( "STRING" ) {
| ^^ can't compare `&Ident` with `str`
|
= help: the trait `std::cmp::PartialEq<str>` is not implemented for `&Ident`
```
`syn::Ident` implements `PartialEq<T> where T: AsRef<str>` so I know I can compare `Ident` with a `str`.
My first reaction was confusion with `str`. It's so rare to see `str` alone that my first instinct was to ensure that what I have is `&str`. While still confused, I decided to throw an `&` in front of my parameter. To my surprise that fixed the error!
... but now I was even more confused. `ident.eq( &"Static string" )` looks a bit weird - and why would it even make a difference?
After a while I realized that my `ident` was a `ref` of `&Ident` - so essentially a double-borrowed `&&Ident`. But as Rust has auto-(de)ref for method calls, this shouldn't matter. I've had `&&&...Foo` types in the past by being overly eager with `&`'s and these have worked just fine without needing any manual `*`'s.
However I've now concluded that the problem here is the built-in `impl PartialEq<&B> for &A`.
With `let ident : &&Ident`, the `ident.eq(..)` gets resolved to:
```rust
impl<'a, 'b, A (&Ident), B = ?>PartialEq<&B> for &A
where A (&Ident) : PartialEq<B> {
fn eq( &self, other: &B ) -> bool;
}
```
Where `B` ends up as `str` to make the `eq(..)` signature match `&str`. Thus the error.
In any case the biggest issue here is the "friendly" error message, I feel: `can't compare '&Ident' with 'str'`. Both types here seem wrong.
- On the right side as far as I can tell, `"STRING"` is `&str`, not `str`.
- On the left side the type should be `&&Ident`, not an `&Ident`.
I guess the parameters are wrong, because Rust does one cycle of derefs. The original types are `&&Ident` and `&str`, but the impl above drops one `&` from each of these.
What purpose does the `PartialEq<&B> for &A` impl serve anyway? If that impl didn't exist, wouldn't rustc do derefs for `&&&A` until it found impl for `A`? Then it would deref the argument. Or is this unwanted behavior in this scenario - even if this is how the auto-deref works everywhere else? Or ~~am I missing~~ I am probably missing some other detail that prevents this from working. | C-enhancement,A-diagnostics,T-compiler | low | Critical |
280,784,791 | pytorch | Make the generator tools data model more explicit | Right now, data is passed around in `gen.py` and similar scripts purely as JSON/YAML, with no schema well-defined at any point. This is bad for developers, who need to essentially reread all of the generator scripts to figure out how any given attribute is being used. Let's make it more explicit. I propose a two pronged approach:
* Named tuples over dictionaries. By pre-declaring the data structures which we fill and pass around, we have an obvious point to hang documentation and format specification
* MyPy for tools only. By adding types, we can machine-check that our structure documentation is up-to-date. MyPy supports a comment syntax which is Python 2 compatible, and is completely optional so non-developers can easily run without any extra installation. | triaged,module: codegen | low | Minor |
280,836,571 | rust | Accessing private fields from the def site of a proc macro | Hi.
I'm not sure whether it is a bug or not, that may be an hygiene issue.
But, in the following [repository](https://github.com/antoyo/proc-macro-private-bug), if you run it with `cargo build`, you get the following error:
```
error[E0451]: field `my_field` of struct `MyStruct` is private
--> src/main.rs:12:5
|
12 | pp!(MyStruct);
| ^^^^^^^^^^^^^^ field `my_field` is private
```
However, that does not make sense, because the field is declared in the [same module](https://github.com/antoyo/proc-macro-private-bug/blob/master/src/main.rs):
```
struct MyStruct {
my_field: i32,
}
fn main() {
pp!(MyStruct); // Error: field `my_field` of struct `MyStruct` is private.
}
```
This only happens when [this feature](https://github.com/antoyo/proc-macro-private-bug/blob/master/Cargo.toml#L10) is enabled.
What makes me think this might be an hygiene issue is that I hard-coded the field name [here](https://github.com/antoyo/proc-macro-private-bug/blob/master/pp/src/lib.rs#L16).
So, if it's a bug, please fix this issue.
Thank you. | T-compiler,A-macros-2.0,C-bug | low | Critical |
280,848,631 | rust | Compiler incorrectly reports function return type declaration as source of inner type mismatch with conservative_impl_trait | Given a function specified as returning a composite type that incorrectly returns an object with an inner type mismatch, the compiler reports the error as being in the function return type declaration rather than at the point the incorrect item is returned.
A sample git repo can be found here: https://git.neosmart.net/mqudsi/futuretest/src/rust-46644
The code in question:
```rust
fn create_future() -> impl Future<Item=(), Error=()> {
return future::ok(Ok(()));
}
```
The compiler returns the following;
```rust
Compiling futuretest v0.1.0 (file:///mnt/d/GIT/futuretest)
error[E0271]: type mismatch resolving `<futures::FutureResult<std::result::Result<(), _>, ()> as futures::Future>::Item == ()`
--> src/main.rs:17:23
|
17 | fn create_future() -> impl Future<Item=(), Error=()> {
| ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ expected enum `std::result::Result`, found ()
|
= note: expected type `std::result::Result<(), _>`
found type `()`
= note: the return type of a function must have a statically known size
error: aborting due to previous error
error: Could not compile `futuretest`.
To learn more, run the command again with --verbose.
```
| C-enhancement,A-diagnostics,T-compiler,A-impl-trait,D-confusing | low | Critical |
280,850,253 | rust | Unsatisfied bounds in case of earlier _inner_ type mismatch error | In case of a type mismatch when chaining/mapping between a `FutureResult<x,y>` and `FutureResult<x,z>`, the compiler also emits (invalid) errors about unsatisfied bounds for any subsequent future operations, even though the type mismatch is only the inner type within the `FutureResult<_,_>` (i.e. in all cases the result of the operation is a future).
Here's an example ([git link](https://git.neosmart.net/mqudsi/futuretest/src/futures-rs-676)):
```rust
let f = future::result::<(),()>(Ok(()))
.map_err(|_| "&'static str error")
.and_then(|_|
future::result(Ok(())
.map_err(|()| "String error".to_owned())
)
)
.and_then(|_| future::result::<(), String>(Err("another &'static str error".to_owned())))
```
generating the following errors, the second of which is the point of this issue:
```rust
Compiling futuretest v0.1.0 (file:///mnt/c/Users/Mahmoud/git/futuretest)
error[E0271]: type mismatch resolving `<futures::FutureResult<(), std::string::String> as futures::IntoFuture>::Error == &str`
--> src/main.rs:12:10
|
12 | .and_then(|_|
| ^^^^^^^^ expected struct `std::string::String`, found &str
|
= note: expected type `std::string::String`
found type `&str`
error[E0599]: no method named `and_then` found for type `futures::AndThen<futures::MapErr<futures::FutureResult<(), ()>, [closure@src/main.rs:11:18: 11:42]>, futures::FutureResult<(), std::string::String>, [closure@src/main.rs:12:19: 15:14]>` in the current scope
--> src/main.rs:17:10
|
17 | .and_then(|_| future::result::<(), String>(Err("another &'static str error".to_owned())))
| ^^^^^^^^
|
= note: the method `and_then` exists but the following trait bounds were not satisfied:
`futures::AndThen<futures::MapErr<futures::FutureResult<(), ()>, [closure@src/main.rs:11:18: 11:42]>, futures::FutureResult<(), std::string::String>, [closure@src/main.rs:12:19: 15:14]> : futures::Future`
`&mut futures::AndThen<futures::MapErr<futures::FutureResult<(), ()>, [closure@src/main.rs:11:18: 11:42]>, futures::FutureResult<(), std::string::String>, [closure@src/main.rs:12:19: 15:14]> : futures::Future`
error: aborting due to 2 previous errors
error: Could not compile `futuretest`.
To learn more, run the command again with --verbose.
```
Resolving the type mismatch fixes the first error and makes the second error go away entirely.
As the trait is implemented regardless of the inner type, it should be possible for the compiler to detect that the second error is not real. | C-enhancement,A-diagnostics,T-compiler | low | Critical |
280,870,883 | opencv | errors and warnings in Opencv 3.3.1 compiled by gcc 7.2 | we cross-compile opencv 3.3.1 using gcc 7.2 , some warnings and errors occur:
the warning:
/home/njai/Downloads/gcc720/arm-buildroot-linux-uclibcgnueabihf/include/c++/7.2.0/bits/stl_algo.h:1940:5: note: parameter passing for argument of type ‘__gnu_cxx::__normal_iterator<cv::ml::PairDI*, std::vector<cv::ml::PairDI> >’ changed in GCC 7.1
/home/njai/Downloads/gcc720/arm-buildroot-linux-uclibcgnueabihf/include/c++/7.2.0/bits/stl_algo.h:1940:5: note: parameter passing for argument of type ‘__gnu_cxx::__normal_iterator<cv::ml::PairDI*, std::vector<cv::ml::PairDI> >’ changed in GCC 7.1
/home/njai/Downloads/gcc720/arm-buildroot-linux-uclibcgnueabihf/include/c++/7.2.0/bits/stl_algo.h:1954:25: note: parameter passing for argument of type ‘__gnu_cxx::__normal_iterator<cv::ml::PairDI*, std::vector<cv::ml::PairDI> >’ changed in GCC 7.1
std::__introsort_loop(__cut, __last, __depth_limit, __comp);
the errors:
/home/njai/Downloads/opencv331/opencv-3.3.1/opencv-3.3.1/modules/ts/include/opencv2/ts/ts_gtest.h:10742:48: error: ‘wstring’ in namespace ‘std’ does not name a type
GTEST_API_ void PrintWideStringTo(const ::std::wstring&s, ::std::ostream* os);
Maybe the error comes from the g++ configure or something else, I am not sure. the warning seems coming from gcc 7.1(or above) change . | category: build/install,incomplete | low | Critical |
280,888,853 | rust | Avoid O-notation for String operations | A couple of places in the `String` docs states that an operation is `O(n)`, usually when discussing a method that needs to shift elements over. However, this is not helpful to users who do not know O-notation, and `n` is generally either misleading or undefined.
[`String::insert_str`](https://doc.rust-lang.org/std/string/struct.String.html#method.insert_str): The operation is really `O(n-idx+k)`, where `n` is the length of `self`, and `k` is the length of `string`. In fact, that's not even true if the String needs to be resized. If we wanted to cover that, we'd need to include the "amortized" qualifier in there. `O(n)` is insufficient in part because if `k` is large and `n` is small, `k` is much more important. We probably want to ditch the O-notation here entirely, and just explain that it needs to shift everything after `idx` over, and copy in the characters from `string`, and that this operation may be slow because of it.
[`String::insert`](https://doc.rust-lang.org/std/string/struct.String.html#method.insert) and [`String::remove`](https://doc.rust-lang.org/std/string/struct.String.html#method.remove): These are less egregious since `O(n)` is correct. However, the smaller bound of `O(n-idx)` can be given, and the difference might be significant. Here too, it'd be better to just get rid of the O-notation altogether and explain what happens instead. | C-enhancement,T-libs-api,A-docs | low | Major |
280,899,724 | go | cmd/go: get should always send go-get parameter, even after redirects | ### What version of Go are you using (`go version`)?
`go version go1.9.2 linux/amd64`
### Does this issue reproduce with the latest release?
:white_check_mark:
### What operating system and processor architecture are you using (`go env`)?
```
GOARCH="amd64"
GOBIN=""
GOEXE=""
GOHOSTARCH="amd64"
GOHOSTOS="linux"
GOOS="linux"
GOPATH="/home/terin/go"
GORACE=""
GOROOT="/usr/lib/go"
GOTOOLDIR="/usr/lib/go/pkg/tool/linux_amd64"
GCCGO="gccgo"
CC="gcc"
GOGCCFLAGS="-fPIC -m64 -pthread -fmessage-length=0 -fdebug-prefix-map=/tmp/go-build604286265=/tmp/go-build -gno-record-gcc-switches"
CXX="g++"
CGO_ENABLED="1"
CGO_CFLAGS="-g -O2"
CGO_CPPFLAGS=""
CGO_CXXFLAGS="-g -O2"
CGO_FFLAGS="-g -O2"
CGO_LDFLAGS="-g -O2"
PKG_CONFIG="pkg-config"
```
### What did you do?
Ran `go get -v -insecure example.org/pkg/foo` whilst "example.org" was directed to a local HTTP server (via host file).
### What did you expect to see?
Code downloaded from a VCS at $GOPATH/src/example.org/pkg/foo.
### What did you see instead?
```
Fetching https://example.org/pkg/foo?go-get=1
https fetch failed: Get https://example.org/pkg/foo?go-get=1: http: server gave HTTP response to HTTPS client
Fetching http://example.org/pkg/foo?go-get=1
Parsing meta tags from http://example.org/pkg/foo?go-get=1 (status code 500)
package example.org/pkg/foo: unrecognized import path "example.org/pkg/foo" (parse http://example.org/pkg/foo?go-get=1: no go-import meta tags ())
```
The behavior of the server is as follows:
* If the request method is not GET or HEAD, responds with a 405 Method Not Allowed
* If the request URL path does not end in a slash, it responds with a 301 Moved Permanently to the URL path with the slash appended
* If the request URL contains the query parameter "go-get=1", it responds with a basic HTTP page with the go-imports meta tag
* If the request URL does not contain the query parameter, it responds with a 303 See Other redirect to godoc.org
Due to an issue in the server's HTTP muxer implementation[^1], the query parameters during the 301 redirect are lost. `go get` dutifully follows the redirect, and as the request no longer contains the "go-get" parameter, it gets and follows the redirection to godoc.org, which responds with a 500 HTTP error.
This seems to be allowed behavior from the HTTP specification; the server should include the query parameters in the Location header to preserve semantics. However, I still found the behavior of `go get` surprising, both that it will sends requests without the "go-get" query parameter, and that it silently follows redirects off the import path domain.
I propose change the behavior of `go get` to always include the "go-get" query parameter, even after redirects, and to log the redirections when the "-v" flag is provided.
---
[^1]: Fortunately, it looks like that HTTP muxer is getting fixed sometime soon [CL#61210](https://go-review.googlesource.com/c/go/+/61210). Now that this author knows about it, he can work around it. | NeedsFix | low | Critical |
281,014,071 | react | React does not call onBlur callback | **Do you want to request a *feature* or report a *bug*?**
**Bug**
**What is the current behavior?**
When input control becomes disabled, React does not call onBlur callback
**If the current behavior is a bug, please provide the steps to reproduce and if possible a minimal https://jsfiddle.net/c22pez5z/
**What is the expected behavior?**
1. Focus on input element
2. Press Enter button (it makes input disabled)
3. `Blur counter` and `Native blur counter` should be equal. | Type: Bug,Component: DOM | low | Critical |
281,063,228 | youtube-dl | Add Support for NFL Gamepass | ## Please follow the guide below
- You will be asked some questions and requested to provide some information, please read them **carefully** and answer honestly
- Put an `x` into all the boxes [ ] relevant to your *issue* (like this: `[x]`)
- Use the *Preview* tab to see what your issue will actually look like
---
### Make sure you are using the *latest* version: run `youtube-dl --version` and ensure your version is *2017.12.10*. If it's not, read [this FAQ entry](https://github.com/rg3/youtube-dl/blob/master/README.md#how-do-i-update-youtube-dl) and update. Issues with outdated version will be rejected.
- [x] I've **verified** and **I assure** that I'm running youtube-dl **2017.12.10**
### Before submitting an *issue* make sure you have:
- [x] At least skimmed through the [README](https://github.com/rg3/youtube-dl/blob/master/README.md), **most notably** the [FAQ](https://github.com/rg3/youtube-dl#faq) and [BUGS](https://github.com/rg3/youtube-dl#bugs) sections
- [x] [Searched](https://github.com/rg3/youtube-dl/search?type=Issues) the bugtracker for similar issues including closed ones
### What is the purpose of your *issue*?
- [ ] Bug report (encountered problems with youtube-dl)
- [x] Site support request (request for adding support for a new site)
- [ ] Feature request (request for a new functionality)
- [ ] Question
- [ ] Other
---
---
### If the purpose of this *issue* is a *bug report*, *site support request* or you are not completely sure provide the full verbose output as follows:
Add the `-v` flag to **your command line** you run youtube-dl with (`youtube-dl -v <your command line>`), copy the **whole** output and insert it here. It should look similar to one below (replace it with **your** log inserted between triple ```):
```
$ youtube-dl --cookies cookies.txt -v -F https://gamepass.nfl.com/game/colts-at-bills-on-12102017
[debug] System config: []
[debug] User config: []
[debug] Custom config: []
[debug] Command-line args: [u'--cookies', u'/Users/maxyellen/Downloads/cookies.txt', u'-v', u'-F', u'https://gamepass.nfl.com/game/colts-at-bills-on-12102017']
[debug] Encodings: locale UTF-8, fs utf-8, out UTF-8, pref UTF-8
[debug] youtube-dl version 2017.12.10
[debug] Python version 2.7.10 - Darwin-17.2.0-x86_64-i386-64bit
[debug] exe versions: ffmpeg 3.4.1, ffprobe 3.4.1
[debug] Proxy map: {}
[generic] colts-at-bills-on-12102017: Requesting header
WARNING: Falling back on generic information extractor.
[generic] colts-at-bills-on-12102017: Downloading webpage
[generic] colts-at-bills-on-12102017: Extracting information
ERROR: Unsupported URL: https://gamepass.nfl.com/game/colts-at-bills-on-12102017
Traceback (most recent call last):
File "/usr/local/bin/youtube-dl/youtube_dl/extractor/generic.py", line 2163, in _real_extract
doc = compat_etree_fromstring(webpage.encode('utf-8'))
File "/usr/local/bin/youtube-dl/youtube_dl/compat.py", line 2539, in compat_etree_fromstring
doc = _XML(text, parser=etree.XMLParser(target=_TreeBuilder(element_factory=_element_factory)))
File "/usr/local/bin/youtube-dl/youtube_dl/compat.py", line 2528, in _XML
parser.feed(text)
File "/System/Library/Frameworks/Python.framework/Versions/2.7/lib/python2.7/xml/etree/ElementTree.py", line 1642, in feed
self._raiseerror(v)
File "/System/Library/Frameworks/Python.framework/Versions/2.7/lib/python2.7/xml/etree/ElementTree.py", line 1506, in _raiseerror
raise err
ParseError: not well-formed (invalid token): line 147, column 31
Traceback (most recent call last):
File "/usr/local/bin/youtube-dl/youtube_dl/YoutubeDL.py", line 784, in extract_info
ie_result = ie.extract(url)
File "/usr/local/bin/youtube-dl/youtube_dl/extractor/common.py", line 437, in extract
ie_result = self._real_extract(url)
File "/usr/local/bin/youtube-dl/youtube_dl/extractor/generic.py", line 3063, in _real_extract
raise UnsupportedError(url)
UnsupportedError: Unsupported URL: https://gamepass.nfl.com/game/colts-at-bills-on-12102017
```
---
### If the purpose of this *issue* is a *site support request* please provide all kinds of example URLs support for which should be included (replace following example URLs by **yours**):
- Single video: https://gamepass.nfl.com/game/colts-at-bills-on-12102017
- Single video: https://gamepass.nfl.com/game/vikings-at-panthers-on-12102017?condensed=true
Note that **youtube-dl does not support sites dedicated to [copyright infringement](https://github.com/rg3/youtube-dl#can-you-add-support-for-this-anime-video-site-or-site-which-shows-current-movies-for-free)**. In order for site support request to be accepted all provided example URLs should not violate any copyrights.
---
### Description of your *issue*, suggested solution and other information
You need a login to access nfl gamepass. I can provide a cookies.txt file, but I don't want to post it publicly. I can email it to you. | account-needed | low | Critical |
281,070,264 | puppeteer | fullPage screenshot duplicates page (doubles/tripples page length) | So I'm having a weird problem with certain websites that I am trying to screenshoot. Essentially the page is shot and then replicated a number of times down in the png to make a really long screenshot that contains all these replications. Its like somebody copy pasted the page a couple of times onto the bottom of the original.
I have not been able to figure out which sites cause it, but the example below is an example- I can supply more if needed.
### Steps to reproduce
**Tell us about your environment:**
* Puppeteer version: 0.13.0
* Platform / OS version: Ubuntu 17.04
* URLs (if applicable): https://bonobos.com/shop/tops
**What steps will reproduce the problem?**
Sample code with fullPage=True, i.e:
```
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://bonobos.com/shop/tops');
await page.screenshot({path: 'example.png', fullPage: true});
await browser.close();
})();
```
**What is the expected result?**
A pull page screenshot- not just the visible part
**What happens instead?**
A very long image that contains multiple copies of the full page screenshot.
<details>

Don't think the example will be visible, but worth a shot.
</details> | bug,upstream,chromium,confirmed,P3 | high | Critical |
281,099,292 | rust | missing_debug_implementations lint is ignored for extern_types | ```Rust
#![feature(extern_types)]
#![warn(missing_debug_implementations)]
pub enum Foo {}
extern "C" {
pub type Bar;
}
```
`Foo` gets a warning, but `Bar` doesn't:
```
warning: type does not implement `fmt::Debug`; consider adding #[derive(Debug)] or a manual implementation
--> foo.rs:5:1
|
5 | pub enum Foo {}
| ^^^^^^^^^^^^^^^
|
note: lint level defined here
--> foo.rs:3:9
|
3 | #![warn(missing_debug_implementations)]
|
``` | A-lints,C-bug,F-extern_types | low | Critical |
281,114,287 | TypeScript | Unable to destructure TypedArrays | **TypeScript Version:** 2.6.2
**Code**
```ts
const [ foo, bar ] = new Float64Array([42, -42])
```
**Expected behavior:**
`foo` and `bar` are destructured correctly
All JS engine with support for TypedArrays and destructuring handles this code without any problem
**Actual behavior:**
Error:` error TS2461: Type 'Float64Array' is not an array type.`
| Suggestion,Committed | low | Critical |
281,158,384 | pytorch | Use the int64 version of MKL calls | then if MKL is present, we can ~~remove~~ avoid the inefficient code in https://github.com/pytorch/pytorch/blob/master/aten/src/TH/generic/THBlas.c .
cc @ezyang @bhosmer @smessmer @ljk53 | module: internals,triaged,module: mkl | low | Minor |
281,166,100 | vscode | Minimap Enhancement: Show Preview Tooltip | Using VS Code 1.18.1
[This is an idea for a new feature, not an issue]
Visual Studio's enhanced scrollbar allows you to hover the minimap and get a full sized preview of the 7-8 lines under your cursor. I would love to see this feature added to the minimap in VS Code. Or perhaps, as some others have suggested, an API for the minimap so extension developers could add this functionality.
Thanks!
Here's what it looks like in VS2017.

| feature-request,editor-minimap | high | Critical |
281,173,227 | rust | allow "NO NOTE" annotations, stop requiring NOTE annotations to be exhaustive | From https://github.com/rust-lang/rust/pull/46641:
I've been very much enjoying the fact that UI tests check for ERRORs. I like being able to add minimal annotations to the test that give the kind of "overall parameters" for what the test is testing (that errors occur here, here, and here) and then having the stderr files capture the full behavior.
But there are times when I want to document a subset of the NOTEs that are present. i.e., so that I can outline "other variations in the stderr output may be acceptable, but if this NOTE disappears, that's a big problem". Note that I say subset -- often there are many notes, but it's just one piece I want to guarantee is present.
Before, we required that if you document any note, you must document all notes. The result is that it discourages me from annotating these notes that I want to be present.
(On the other hand, if you can't declare that the set of notes is exhaustive, you can't document that a note should not appear. I don't find this to be something I want to document very often, but it's certainly possible. But we could easily have a "NO_NOTE" annotation or something then.)
What I do for now is to leave comments, but I fear that future people will not read them.
cc @oli-obk @petrochenkov @estebank | A-testsuite,E-hard,C-enhancement,T-bootstrap,A-contributor-roadblock,A-compiletest,E-needs-design | low | Critical |
281,201,955 | go | go/types: support cgo semantics | I was thinking about https://github.com/golang/go/issues/975.
My idea is guessing argument types and making specialized wrappers.
For example,
We can convert following code
```
package main
// #include <stdio.h>
import "C"
func main() {
C.printf(C.CString("hello: %d"), 10)
C.fflush(C.stdout)
}
```
into
```
package main
// #include <stdio.h>
// int call_printf_with_int(const char* s, int i) {
// return printf(s, i);
// }
import "C"
func main() {
C.call_printf_with_int(C.CString("hello: %d"), 10)
C.fflush(C.stdout)
}
```
I'd like to leave these translations to cgo.
To promote this idea, we have to make `go/types` understand some special cgo semantics.
* all cgo objects are exportable even if the first letter is lower case (e.g. `C.char`, `C.int`, ...)
* cgo functions behave like `unsafe.Pointer` in the expression context (e.g. `var xxx unsafe.Pointer = C.puts`)
* cgo functions can return the optional `error` argument in the assignment context (e.g `_, err := C.puts(C.CString("x"))`
Is this acceptable?
FWIW, https://go-review.googlesource.com/c/go/+/83215 is the attempt. | NeedsInvestigation,FeatureRequest | low | Critical |
281,205,670 | godot | Changing node parent produces Area2D/3D signal duplicates | Godot 2.1.4, Godot 3.0 beta 1
Windows 10 64 bits
I found that if you teleport-away and reparent a node which is inside an Area2D, you will get an `area_exit`, but ALSO extras notifications `body_enter` and `body_exit`, two in my case, even though the object is not anymore in the area.
This gave me headaches for several hours in my game until I narrow it down to this, because this behavior causes my teleport system to trigger everything multiple times, and I can't find a way to avoid this so far without atrocious hacks...
It does this wether I teleport from `fixed_process` or not, and emptying layer/collision masks before teleport has no effect.
Here is a demo project reproducing it:
2.1.4: [TeleportArea2D.zip](https://github.com/godotengine/godot/files/1549727/TeleportArea2D.zip)
3.0b1: [TeleportArea2D_Godot3b1.zip](https://github.com/godotengine/godot/files/1549767/TeleportArea2D_Godot3b1.zip)
Repro:
1) Launch main scene, notice you get one `body_enter` because the body overlaps with the area, wich is expected
2) Press any key, this teleports the body and reparents it (in code, the node is removed from tree, translated, and then added to the tree)
3) Notice you get an expected `body_exit`, but also two unexpected pairs of `body_enter` and `body_exit` | bug,confirmed,topic:physics | medium | Critical |
281,242,864 | rust | rustc accepts impls that has different type signatures from trait decls in lifetime parameters of the type | I thought this code was invalid because 1. it had a redundant lifetime parameter and 2. it had different types from the trait decl in the types of the argument and the return value.
```rust
trait Foo<T> {
fn foo(&self, t: T) -> T;
}
impl<'a> Foo<Option<&'a str>> for () {
fn foo<'b>(&self, t: Option<&'b str>) -> Option<&'b str> {
t
}
}
```
but the compiler (both stable (1.22.1) and nightly (9fe7aa353 2017-12-11)) accepts this code.
Is this indended or a bug?
FYI: it seems compiler treat the signature method `foo` as the one of `Foo` (or, perhaps unifying `'b` to `'a`?) so it doesn't cause unsafe states like dangling pointer.
```rust
fn main() {
let s = "String".to_string();
{
let o = Some(s.as_ref());
let _ = <() as Foo<Option<&'static str>>>::foo(&(), Some("static"));
// error
let _ = <() as Foo<Option<&'static str>>>::foo(&(), o);
// error
let _: Option<&'static str> = ().foo(o);
}
}
``` | A-lifetimes,A-trait-system,T-compiler,C-bug,T-types | low | Critical |
281,247,967 | rust | Better error reporting for Sync and type that impl !Sync | It would make a lot more sense if when a type `impl !Sync` the compiler could report an other error than the error that comes from [this impl block](https://doc.rust-lang.org/beta/src/core/marker.rs.html#557).
For example, take a look at this code :
```rust
use std::sync::mpsc::channel;
use std::thread;
struct Foo {}
impl Foo {
fn do_something(&self) {
println!("hello");
}
}
fn main() {
let (tx, rx) = channel::<Foo>();
thread::spawn(move || rx.iter().map(|f| f.do_something()));
}
```
Which outputs :
```
17 | thread::spawn(move || rx.iter().map(|f| f.do_something()));
| ^^^^^^^^^^^^^ `std::sync::mpsc::Receiver<Foo>` cannot be shared between threads safely
|
= help: the trait `std::marker::Sync` is not implemented for `std::sync::mpsc::Receiver<Foo>`
= note: required because of the requirements on the impl of `std::marker::Send` for `&std::sync::mpsc::Receiver<Foo>`
= note: required because it appears within the type `std::sync::mpsc::Iter<'_, Foo>`
= note: required because it appears within the type `std::iter::Map<std::sync::mpsc::Iter<'_, Foo>, [closure@src/main.rs:17:41: 17:61]>`
= note: required by `std::thread::spawn`
```
It would be way better if it could just say that `Receiver` is [explicitly](https://doc.rust-lang.org/src/std/sync/mpsc/mod.rs.html#339) not `Sync`.
I know that this a bad example because the error is actually due to the fact that the compiler does not move `rx` because we are returning a value from the call to `map()`, but it was the only one that I had... | C-enhancement,A-diagnostics,A-trait-system,T-compiler | low | Critical |
281,256,778 | go | runtime: delineate multiple panics by adding newlines between them | Please answer these questions before submitting your issue. Thanks!
### What version of Go are you using (`go version`)?
go version go1.9.2 linux/amd64
### What did you do?
```
// a.go
package main
func main() {
var s []int
var is interface{} = s
go func() {
_ = s == nil
}()
go func() {
_ = is == is
}()
var m map[string]bool = nil
_ = m["Go"]
_, _ = m["Go"]
m, _ = is.(map[string]bool)
m = is.(map[string]bool)
}
```
### What did you expect to see?
```
$ go run a.go
panic: interface conversion: interface {} is []int, not map[string]bool
goroutine 1 [running]:
main.main()
/tmp/a.go:17 +0x15a
panic: runtime error: comparing uncomparable type []int
goroutine 19 [running]:
main.main.func2(0x45b480, 0x4cc2c0)
/tmp/a.go:10 +0x3a
created by main.main
/tmp/a.go:9 +0xc9
exit status 2
```
### What did you see instead?
```
$ go run a.go
panic: interface conversion: interface {} is []int, not map[string]bool
goroutine 1 [running]:
main.main()
/tmp/a.go:17 +0x15a
panic: runtime error: comparing uncomparable type []int
goroutine 19 [running]:
main.main.func2(0x45b480, 0x4cc2c0)
/tmp/a.go:10 +0x3a
created by main.main
/tmp/a.go:9 +0xc9
exit status 2
```
| help wanted,NeedsFix | low | Critical |
281,361,191 | ant-design | will the Tabs can be triggered by using hover? | ### What problem does this feature solve?
if Tabs can only be changed by click, it's not cooool
### What does the proposed API look like?
onMouseEnter
onMouseLeave
<!-- generated by ant-design-issue-helper. DO NOT REMOVE --> | 💡 Feature Request,Inactive,IssueHuntFest | low | Minor |
281,369,679 | rust | [E0277] Add Note when trait is not satisfied because of missing reference in where | When "where T: TraitX" is missing an "&" the error message E0277 could be improved with a note.
I'm also not sure why the same looking code (`fn a<B>(b: &B) where B: TraitB;`) inside a trait compiles just fine, maybe there is more to it.
I tried this code:
```rust
trait TraitA { fn a<B>(b: &B) where B: TraitB; }
struct StructA { }
impl TraitA for StructA { fn a<B>(b: &B) where B: TraitB { } }
trait TraitB { fn b(); }
struct StructB { }
impl TraitB for StructB { fn b() { } }
// (should be:) fn c<'a, B: 'a>(b: &'a B) where &'a B: TraitB
fn c<B>(b: &B) where B: TraitB {
StructA::a(&b);
}
```
I expected to see this happen (or similar):
```text
[...]
= note: Try "where &'a B: TraitB" instead of "where B: TraitB"
```
Instead, this happened:
```text
error[E0277]: the trait bound `&B: TraitB` is not satisfied
--> src/lib.rs:20:9
|
20 | StructA::a(&b);
| ^^^^^^^^^^ the trait `TraitB` is not implemented for `&B`
|
= note: required by `TraitA::a`
```
## Meta
`rustc --version --verbose`:
```
rustc 1.24.0-nightly (9fe7aa353 2017-12-11)
binary: rustc
commit-hash: 9fe7aa353fac5084d0a44d6a15970310e9be67f4
commit-date: 2017-12-11
host: x86_64-unknown-linux-gnu
release: 1.24.0-nightly
LLVM version: 4.0
``` | C-enhancement,A-diagnostics,T-compiler | low | Critical |
281,397,594 | svelte | Document the architecture behind Svelte | Hi,
I'm really interested in how svelte internally works and which problems and challenges you had faced. I could read the whole source code but the author can provide a much better picture. Svelte is able to compile itself away this is amazing because when the API is settled you can share performant, runtime-independent web components.
Interesting points
- Code generation
- Compile-time static analysis
- Role of Typescript
- Your motivation
- Roadmap
- .....
| meta,documentation | medium | Critical |
281,401,115 | vscode | Wrap with abbreviation history | Coming from atom this is a feature that i miss.
When wrapping with abbreviation you could use the up and down arrows to go through previously used abbreviations.
So if you had created a long abbreviation and it was slightly wrong or you wanted to reuse you wouldn't have to type it out all over again. | help wanted,feature-request,emmet | low | Major |
281,424,458 | TypeScript | TypeScript VS: Doesn't re-transpile file when source-control revert-changes | TypeScript integration in Visual Studio 2017:
When I change a typescript-file in a visual studio project ( that is in TFS source control )
then I decide to abandon the change (revert changes from right-click context menu)
then Visual Studio correctly replaces the local typescript-file with the server version.
But it doesn't re-transpile the changed file.
`"compileOnSave": true,`
also, the option should then be called/added "compileOnChange", not compileOnSave...
Because apparently, these two things are not the same, which i find somewhat surprising...
although ...
Consequence is, the JavaScript file doesn't run as it should when debugging. | Visual Studio,Needs Investigation | low | Critical |
281,439,851 | vscode | indent back to line start after enter newline and press up | - VSCode Version: Code 1.18.1 (929bacba01ef658b873545e26034d1a8067445e9, 2017-11-16T18:32:36.026Z)
- OS Version: Windows_NT ia32 10.0.16299
Hi, I have a feature request related to intent,
I think Notepad++ and Visual Studio's indent `after enter newline and press up` works better and more intuitive, it'll remain the indent position, as in the gif:
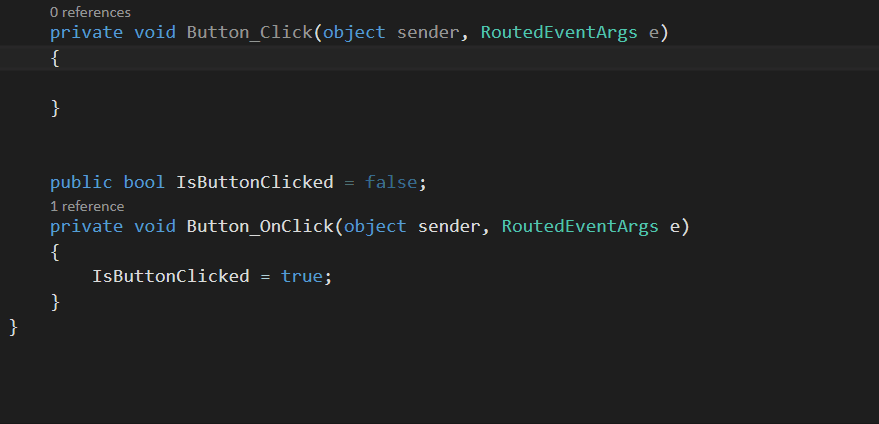
But VSCode's indent `after enter newline and press up` go back to line start, which I think is not so reasonable:
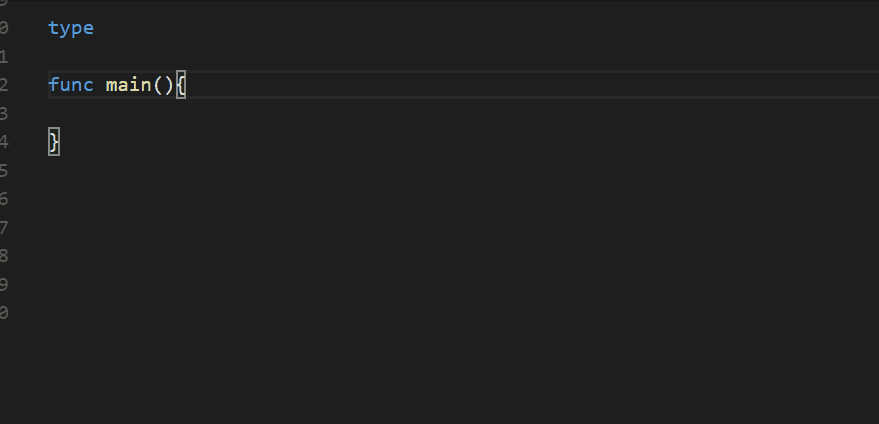
Hope vscode can remain the indent position `after enter newline and press up`, thank you.
.
<!-- Launch with `code --disable-extensions` to check. -->
Reproduces without extensions: Yes | editor-autoindent,under-discussion | high | Critical |
281,450,438 | ant-design | Upload component - enable sending multiple files in one XHR request | ### What problem does this feature solve?
Simplifies back-end implementation when in need to process multiple files at the same time; and when files need to be processed in one transaction without the need for the front-end to have the link to the uploaded files.
### What does the proposed API look like?
It can be similar or the same as jQuery-File-Upload *[singleFileUploads](https://github.com/blueimp/jQuery-File-Upload/wiki/Options#singlefileuploads)* option when the multiple option is enabled on the Upload component.
<!-- generated by ant-design-issue-helper. DO NOT REMOVE --> | help wanted,💡 Feature Request,Inactive,IssueHuntFest | medium | Critical |
281,454,880 | pytorch | x.grad should be 0 but get NaN after x/0 | # x.grad should be 0 but get NaN after x/0
### Reproduction BUG code
```python
import torch
from torch.autograd import Variable
x = Variable(torch.FloatTensor([1.,1]), requires_grad=True)
div = Variable(torch.FloatTensor([0.,1]))
y = x/div # => y is [inf, 1]
zero_mask = (div==0) # => zero_mask is [1, 0]
y[zero_mask] = 0 # => y is [0, 1]
loss = y.sum()
loss.backward()
print(x.grad) # grad is [nan, 1], but expected [0, 1]
```
Computational graph of loss not include `x[0]`
So, gradient of `x[0]` should be 0, but get `NaN`
### more simple reproduction
```python
x = Variable(torch.FloatTensor([1.,1]), requires_grad=True)
div = Variable(torch.FloatTensor([0.,1]))
y = x/div # => y is [inf, 1]
mask = (div!=0) # => mask is [0, 1]
loss = y[mask]
loss.backward()
print(x.grad) # grad is [nan, 1], but expected [0, 1]
```
### Versions:
* Python: 2.7
* pyTorch: 0.3.0.post4
cc @svekars @holly1238 @ezyang @albanD @zou3519 @gqchen @pearu @nikitaved @soulitzer @Lezcano @Varal7 @brianjo @mruberry @gchanan @bdhirsh @jbschlosser @anjali411 @jlin27 | module: docs,module: autograd,triaged,module: NaNs and Infs,has workaround,needs design | medium | Critical |
281,480,621 | flutter | libtxt: TextPainter.minIntrinsicWidth doesn't take into account ellipsis or maxLines | I've only tested this with the Blink text rendering. | engine,a: typography,P2,c: tech-debt,team: skip-test,team-engine,triaged-engine | low | Minor |
281,501,683 | TypeScript | Quick fix to install regular .js npm packages | Let's say I start a new project. I start writing out an import for, say, `express` because I'm certain I'll be using that, but I haven't bothered to install it yet.
```ts
import express from "express";
```
Immediately, I might get an error like `Cannot find module 'express'.` because I didn't install the package before using it. This happens regardless of if I have `noImplicitAny` on. But this means I have to switch to a terminal and do an npm install.
We already give a quick fix to install missing `@types` packages, we should have a fix that
1. Gives the option of installing a missing package as a dependency or a dev dependency.
2. Also installs the types for that package if necessary in the same fashion. | Suggestion,In Discussion,Domain: Quick Fixes | low | Critical |
281,526,095 | rust | Lints sometimes don't trigger, with no discernable pattern | This has cropped up in Diesel for a long time, either manifesting as having to change some unrelated code because a lint started triggering that wasn't before, or occasionally noticing "oh hey you need to implement `Copy` on this type, why didn't the lint trigger?"
I finally have a case that I can report though, which I noticed before it started failing, and very clearly should be triggering. https://github.com/diesel-rs/diesel/pull/1375 adds `#[deny(missing_docs)]` to the `query_dsl` module. However, `query_dsl::boxed_dsl::BoxedDsl` is a public item which has no documentation on it, and should be triggering the lint. I can even go in and add `#[deny(missing_docs)]` to that trait directly, and the lint still doesn't fire.
I'm not sure what other information I can add -- This is not specific to this one lint in this one case, we've had tons of cases of lints which should have always been failing randomly starting to fail due to changes in completely unrelated code. | C-enhancement,A-lints,T-compiler,E-needs-mcve | low | Major |
281,562,137 | rust | arguments `<optimized out>` when optimizations remove unused data fields | This program:
```rust
use std::env;
#[inline(never)]
fn foo(x: &str, y: &str) -> usize {
x.len() + y.len()
}
fn main() {
let mut args = env::args();
let x = args.next().unwrap();
let y = args.next().unwrap();
let z = foo(&x, &y);
println!("z={:?}", z);
}
```
when compiled with optimizations enabled, seems to strip out all of the local variable information:
```
lunch-box. rustc +nightly -O -g ~/tmp/foo.rs
lunch-box. gdb foo
Error in sitecustomize; set PYTHONVERBOSE for traceback:
AttributeError: module 'sys' has no attribute 'setdefaultencoding'
GNU gdb (Ubuntu 7.12.50.20170314-0ubuntu1.1) 7.12.50.20170314-git
Copyright (C) 2017 Free Software Foundation, Inc.
License GPLv3+: GNU GPL version 3 or later <http://gnu.org/licenses/gpl.html>
This is free software: you are free to change and redistribute it.
There is NO WARRANTY, to the extent permitted by law. Type "show copying"
and "show warranty" for details.
This GDB was configured as "x86_64-linux-gnu".
Type "show configuration" for configuration details.
For bug reporting instructions, please see:
<http://www.gnu.org/software/gdb/bugs/>.
Find the GDB manual and other documentation resources online at:
<http://www.gnu.org/software/gdb/documentation/>.
For help, type "help".
Type "apropos word" to search for commands related to "word"...
Reading symbols from foo...done.
warning: Missing auto-load script at offset 0 in section .debug_gdb_scripts
of file /home/nmatsakis/tmp/foo.
Use `info auto-load python-scripts [REGEXP]' to list them.
(gdb) break 3
Breakpoint 1 at 0x7264: file /home/nmatsakis/tmp/foo.rs, line 3.
(gdb) r a b
Starting program: /home/nmatsakis/tmp/foo a b
[Thread debugging using libthread_db enabled]
Using host libthread_db library "/lib/x86_64-linux-gnu/libthread_db.so.1".
Breakpoint 1, foo::foo (x=..., y=...) at /home/nmatsakis/tmp/foo.rs:5
5 x.len() + y.len()
(gdb) p x
$1 = <optimized out>
```
cc @tromey | A-debuginfo,T-compiler,C-bug | low | Critical |
281,587,626 | rust | item paths use the name of the item, which might differ from the name of the import | ## STR
Crate foo:
```Rust
mod internal {
pub struct S;
}
pub use internal::S as T;
```
Crate main:
```Rust
extern crate foo;
fn main() {
foo::T
}
```
## Results
```
error[E0308]: mismatched types
--> x.rs:4:5
|
3 | fn main() {
| - expected `()` because of default return type
4 | foo::T
| ^^^^^^ expected (), found struct `foo::S`
|
= note: expected type `()`
found type `foo::S`
error: aborting due to previous error
```
The error message refers to `foo::S`, which does not exist (only `foo::T` does) | C-enhancement,A-diagnostics,T-compiler | low | Critical |
281,599,578 | rust | Use SIMD to accelerate UTF-8 encoding and decoding | This is a feature request: use SIMD instructions to accelerate UTF-8 encoding and decoding operations. | C-enhancement,T-libs,A-str | low | Minor |
281,611,997 | nvm | nvm install --lts failed |
- Operating system and version:
osx 10.12.6
- `nvm debug` output:
<details>
<!-- do not delete the following blank line -->
```sh
nvm --version: v0.33.0
$SHELL: /bin/bash
$HOME: /Users/ruiguo
$NVM_DIR: '$HOME/.nvm'
$PREFIX: ''
$NPM_CONFIG_PREFIX: ''
nvm current: system
which node: /usr/local/bin/node
which iojs:
which npm: /usr/local/bin/npm
npm config get prefix: /usr/local
npm root -g: /usr/local/lib/node_modules
```
</details>
- `nvm ls` output:
<details>
<!-- do not delete the following blank line -->
```sh
-> system
node -> stable (-> N/A) (default)
iojs -> N/A (default)
grep: brackets ([ ]) not balanced
sed: 8: "
s#/Users/r ...": unbalanced brackets ([])
lts/* -> lts/<![endif]--> (-> N/A)
sed: 8: "
s#/Users/r ...": unbalanced brackets ([])
lts/<![endif]--> -> <!--[if (-> N/A)
sed: 8: "
s#/Users/r ...": unbalanced brackets ([])
lts/><![endif]--> -> <!--[if (-> N/A)
```
</details>
- How did you install `nvm`? (e.g. install script in readme, homebrew):
curl -o- https://raw.githubusercontent.com/creationix/nvm/v0.33.0/install.sh | bash
- What steps did you perform?
`nvm install --lts`
- What happened?
```sh
Installing latest LTS version.
Detected that you have 4 CPU core(s)
Running with 3 threads to speed up the build
grep: brackets ([ ]) not balanced
Downloading https://nodejs.org/dist/node-<!--[if.tar.gz...
curl: (3) [globbing] bad range in column 35
Binary download from https://nodejs.org/dist/node-<!--[if.tar.gz failed, trying source.
grep: /Users/ruiguo/.nvm/.cache/src/node-<!--[if/node-<!--[if.tar.gz: No such file or directory
Provided file to checksum does not exist.
nvm: install <!--[if failed!
```
- What did you expect to happen?
How to install?
- Is there anything in any of your profile files (`.bashrc`, `.bash_profile`, `.zshrc`, etc) that modifies the `PATH`?
I think that does not matter
<!-- if this does not apply, please delete this section -->
- If you are having installation issues, or getting "N/A", what does `curl -I --compressed -v https://nodejs.org/dist/` print out?
<details>
<!-- do not delete the following blank line -->
```sh
curl -I --compressed -v https://nodejs.org/dist/
* Trying 104.20.22.46...
* TCP_NODELAY set
* Trying 2400:cb00:2048:1::6814:172e...
* TCP_NODELAY set
* Immediate connect fail for 2400:cb00:2048:1::6814:172e: No route to host
* Trying 2400:cb00:2048:1::6814:162e...
* TCP_NODELAY set
* Immediate connect fail for 2400:cb00:2048:1::6814:162e: No route to host
* Connected to nodejs.org (104.20.22.46) port 443 (#0)
* TLS 1.2 connection using TLS_ECDHE_RSA_WITH_AES_128_GCM_SHA256
* Server certificate: *.nodejs.org
* Server certificate: COMODO RSA Domain Validation Secure Server CA
* Server certificate: COMODO RSA Certification Authority
> HEAD /dist/ HTTP/1.1
> Host: nodejs.org
> User-Agent: curl/7.54.0
> Accept: */*
> Accept-Encoding: deflate, gzip
>
< HTTP/1.1 403 Forbidden
HTTP/1.1 403 Forbidden
< Date: Wed, 13 Dec 2017 03:15:50 GMT
Date: Wed, 13 Dec 2017 03:15:50 GMT
< Content-Type: text/html; charset=UTF-8
Content-Type: text/html; charset=UTF-8
< Connection: close
Connection: close
< Set-Cookie: __cfduid=d4f20af1753e377233a26c51bb06607dd1513134950; expires=Thu, 13-Dec-18 03:15:50 GMT; path=/; domain=.nodejs.org; HttpOnly
Set-Cookie: __cfduid=d4f20af1753e377233a26c51bb06607dd1513134950; expires=Thu, 13-Dec-18 03:15:50 GMT; path=/; domain=.nodejs.org; HttpOnly
< CF-Chl-Bypass: 1
CF-Chl-Bypass: 1
< Cache-Control: max-age=2
Cache-Control: max-age=2
< Expires: Wed, 13 Dec 2017 03:15:52 GMT
Expires: Wed, 13 Dec 2017 03:15:52 GMT
< X-Frame-Options: SAMEORIGIN
X-Frame-Options: SAMEORIGIN
< Server: cloudflare-nginx
Server: cloudflare-nginx
< CF-RAY: 3cc5c2debc9b2876-SJC
CF-RAY: 3cc5c2debc9b2876-SJC
<
* Closing connection 0
```
</details> | shell: bash/sh,OS: Mac OS,needs followup | medium | Critical |
281,620,749 | rust | If specialization is involved, associated types are not evaluated even on last crate. | As current specialization does not support returning `default type XX` from default method (so it can be specialized separately), I used a trick to do this.
Playground: https://play.rust-lang.org/?gist=d4bbcd09ae0cd3044d2da9879fc489a0&version=nightly
It compiles, and I expected it to work, but I noticed that it doesn't work because associated type isn't evaluated.
```sh
error[E0369]: binary operation `==` cannot be applied to type `<<{integer} as Specializer>::Specialized as SpecialzedTrait>::Out`
--> src/main.rs:72:5
|
72 | assert_eq!(1.op(), (1, 2).op());
| ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
|
= note: an implementation of `std::cmp::PartialEq` might be missing for `<<{integer} as Specializer>::Specialized as SpecialzedTrait>::Out`
= note: this error originates in a macro outside of the current crate (in Nightly builds, run with -Z external-macro-backtrace for more info)
error[E0277]: the trait bound `<<{integer} as Specializer>::Specialized as SpecialzedTrait>::Out: std::fmt::Debug` is not satisfied
--> src/main.rs:72:5
|
72 | assert_eq!(1.op(), (1, 2).op());
| ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ `<<{integer} as Specializer>::Specialized as SpecialzedTrait>::Out` cannot be formatted using `:?`; if it is defined in your crate, add `#[derive(Debug)]` or manually implement it
|
= help: the trait `std::fmt::Debug` is not implemented for `<<{integer} as Specializer>::Specialized as SpecialzedTrait>::Out`
= note: required because of the requirements on the impl of `std::fmt::Debug` for `&<<{integer} as Specializer>::Specialized as SpecialzedTrait>::Out`
= note: required by `std::fmt::Debug::fmt`
= note: this error originates in a macro outside of the current crate (in Nightly builds, run with -Z external-macro-backtrace for more info)
error[E0277]: the trait bound `{integer}: std::cmp::PartialEq<<<{integer} as Specializer>::Specialized as SpecialzedTrait>::Out>` is not satisfied
--> src/main.rs:73:5
|
73 | assert_eq!((1, 2).op(), 1.op());
| ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ can't compare `{integer}` with `<<{integer} as Specializer>::Specialized as SpecialzedTrait>::Out`
|
= help: the trait `std::cmp::PartialEq<<<{integer} as Specializer>::Specialized as SpecialzedTrait>::Out>` is not implemented for `{integer}`
= note: this error originates in a macro outside of the current crate (in Nightly builds, run with -Z external-macro-backtrace for more info)
error[E0277]: the trait bound `<<{integer} as Specializer>::Specialized as SpecialzedTrait>::Out: std::fmt::Debug` is not satisfied
--> src/main.rs:73:5
|
73 | assert_eq!((1, 2).op(), 1.op());
| ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ `<<{integer} as Specializer>::Specialized as SpecialzedTrait>::Out` cannot be formatted using `:?`; if it is defined in your crate, add `#[derive(Debug)]` or manually implement it
|
= help: the trait `std::fmt::Debug` is not implemented for `<<{integer} as Specializer>::Specialized as SpecialzedTrait>::Out`
= note: required because of the requirements on the impl of `std::fmt::Debug` for `&<<{integer} as Specializer>::Specialized as SpecialzedTrait>::Out`
= note: required by `std::fmt::Debug::fmt`
= note: this error originates in a macro outside of the current crate (in Nightly builds, run with -Z external-macro-backtrace for more info)
error: aborting due to 4 previous errors
error: Could not compile `playground`.
To learn more, run the command again with --verbose.
```
I assume it's not evaluated because rustc thinks it *can* be specialized. But it can't be, because
- it's last crate
- it's not public
To make it not specializable, I tried auto trait like https://play.rust-lang.org/?gist=d4bbcd09ae0cd3044d2da9879fc489a0&version=nightly , but it doesn't work because `impl<A, B> !NotTuple for (A, B) {}` implies `!NotTuple` for any struct with `(A, B)`.
```sh
error[E0277]: the trait bound `T: NotTuple` is not satisfied
--> src\main.rs:80:9
|
80 | impl<T> AssertAll for T {}
| ^^^^^^^^^ the trait `NotTuple` is not implemented for `T`
|
= help: consider adding a `where T: NotTuple` bound
= note: required because of the requirements on the impl of `Specializer` for `T`
= note: required because of the requirements on the impl of `MyTrait` for `T`
error: aborting due to previous error
error: Could not compile `issue`.
To learn more, run the command again with --verbose.
```
| T-compiler,A-specialization,C-bug,F-specialization | low | Critical |
281,623,439 | go | proposal: add golang.org/x/text/diff package | Many test code are something like:
```
func TestAAA(t *testing.T) {
if got != want {
t.Errorf("got %v, want %v")
}
}
```
However, these kind of code are pretty useless when we expected multi-line values.
For example,
```
--- FAIL: TestExamples (0.00s)
example_test.go:186: Import: got Play == "package main\n\nimport (\n\t\"fmt\"\n\t\"log\"\n\t\"os/exec\"\n)\n\nfunc main() {\n\tout, err := exec.Command(\"date\").Output()\n\tif err != nil {\n\t\tlog.Fatal(err)\n\t}\n\tfmt.Printf(\"The date is %s\\n\", out)\n}\n", want "package main\n\nimport (\n\t\"fmt\"\n\t\"log\"\n\t\"os/exec\"\n)\n\n// aaa\n\nfunc main() {\n out, err := exec.Command(\"date\").Output()\n\tif err != nil {\n\t\tlog.Fatal(err)\n\t}\n\tfmt.Printf(\"The date is %s\\n\", out)\n}\n"
FAIL
```
So, I propose to support diff algorithm for making error messages human-readable.
The simplest implementation is executing the `diff` command. It doesn't work on windows.
But we can use fallback, so it can be a good starting point.
I'm not sure that supporting the "diff" package officially is worth doing.
But "internal" packages seem harmless. | Proposal,Proposal-Hold | low | Critical |
281,629,111 | go | x/image/tiff: no support for cJPEG or cJPEGOld | #### What did you do?
tried to decode a jpeg compressed tiff or a canon raw (weird tiff with jpeg data)
#### What did you expect to see?
the image to be decoded correctly without errors.
#### What did you see instead?
with a cr2:
`tiff: unsupported feature: compression value 6`
with a tiff with proper jpeg compressed image data:
`tiff: unsupported feature: compression value 7`
#### System details
```
go version go1.9.2 linux/amd64
GOARCH="amd64"
GOBIN=""
GOEXE=""
GOHOSTARCH="amd64"
GOHOSTOS="linux"
GOOS="linux"
GOPATH="/home/gareth/go"
GORACE=""
GOROOT="/usr/lib/go"
GOTOOLDIR="/usr/lib/go/pkg/tool/linux_amd64"
GCCGO="gccgo"
CC="gcc"
GOGCCFLAGS="-fPIC -m64 -pthread -fmessage-length=0 -fdebug-prefix-map=/tmp/go-build053335415=/tmp/go-build -gno-record-gcc-switches"
CXX="g++"
CGO_ENABLED="1"
CGO_CFLAGS="-g -O2"
CGO_CPPFLAGS=""
CGO_CXXFLAGS="-g -O2"
CGO_FFLAGS="-g -O2"
CGO_LDFLAGS="-g -O2"
PKG_CONFIG="pkg-config"
GOROOT/bin/go version: go version go1.9.2 linux/amd64
GOROOT/bin/go tool compile -V: compile version go1.9.2
uname -sr: Linux 4.14.4-1-ARCH
LSB Version: 1.4
Distributor ID: Arch
Description: Arch Linux
Release: rolling
Codename: n/a
/usr/lib/libc.so.6: GNU C Library (GNU libc) stable release version 2.26, by Roland McGrath et al.
gdb --version: GNU gdb (GDB) 8.0.1
```
| NeedsInvestigation | low | Critical |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.