repo_name
stringlengths 6
91
| path
stringlengths 8
968
| copies
stringclasses 210
values | size
stringlengths 2
7
| content
stringlengths 61
1.01M
| license
stringclasses 15
values | hash
stringlengths 32
32
| line_mean
float64 6
99.8
| line_max
int64 12
1k
| alpha_frac
float64 0.3
0.91
| ratio
float64 2
9.89
| autogenerated
bool 1
class | config_or_test
bool 2
classes | has_no_keywords
bool 2
classes | has_few_assignments
bool 1
class |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
anupharbade/Playground | Swift.playground/Pages/CopyOnWrite.xcplaygroundpage/Contents.swift | 1 | 1995 | //: [Previous](@previous)
import Foundation
var str = "Hello, playground"
func address(of object: UnsafeRawPointer) -> String {
let addr = Int(bitPattern: object)
return String(format: "%p", addr)
}
var array = [1,2,3,4]
var copiedArray = array
//Both should have same address in the memory.
//This is because Array handles copy differently.
//The copy mechanism that array follows is copy on write.
//This mechanism copies the instances only when the instance is being mutated.
//Mostly this is to acheive performance
address(of:array)
address(of:copiedArray)
array.append(5)
//Here since we are trying to mutate the array it should create the copy of the array.
//This address should be different.
address(of:array)
copiedArray.append(5)
//Just bcz copiedArray was assigned with the original array at the time of assignment, Swift must be maintaining it as a
//copied instance which must be copied on write.
//We are trying to modify the instance here and hence in this case we need a separate instance as per the value type paradigm.
//CopiedArray will be copied to some other location now and must have different address than the previous.
//ARC must be taking care of releasing the previously allocated address.
address(of:copiedArray)
//We are trying to assign the copiedArray to array back.
//In which case the previous array instance should be released and the copiedArray address should be assigned.
//Printing the address of both the vars should print the same addresses
array = copiedArray
address(of:array)
address(of:copiedArray)
//Here the copiedArray is being mutated again and hence as per copy on write principle, it should be copied and assigned a new address
copiedArray.remove(at: 4)
address(of:copiedArray)
//array should have the same address as it had during assignment.
address(of: array)
//Trying to mutate array should again copy the address to another location and have the different address.
array.append(6)
address(of: array)
//: [Next](@next)
| mit | 2735a58c233c24ed968f6786a041b5d6 | 34.625 | 134 | 0.773434 | 4.030303 | false | false | false | false |
IBM-MIL/IBM-Ready-App-for-Insurance | PerchReadyApp/apps/Perch/iphone/native/Perch/Controllers/AssetsViewController.swift | 1 | 9876 | /*
Licensed Materials - Property of IBM
© Copyright IBM Corporation 2015. All Rights Reserved.
*/
import UIKit
import QuartzCore
/**
* View Controller to display a user's different home sensor assets that are connected with the app
*/
class AssetsViewController: PerchViewController {
@IBOutlet weak var assetCollectionView: UICollectionView!
/// edge insets for all iPhones, iPad has more padding
let standardEdgeInsets = UIEdgeInsetsMake(8, 20, 0, 20)
/// Boolean checked when a new notification has come in
var isVisible = false
weak var navDelegate: NavHandlerDelegate?
let perchAlertManager = PerchAlertViewManager.sharedInstance
let assetOverviewDataManager = AssetOverviewDataManager.sharedInstance
// MARK: Lifecycle
override func viewDidLoad() {
super.viewDidLoad()
// No need to query asset data on view did load. Asset data should have been queried from Pin VC to get to this point
}
override func viewDidAppear(animated: Bool) {
super.viewDidAppear(animated)
isVisible = true
// Make sure to set the callback in case it has been changed elsewhere
self.assetOverviewDataManager.callback = {[unowned self] in self.assetDataReturned($0)}
if AssetOverviewDataManager.sharedInstance.shouldReload {
getAllAssetData()
} else {
assetCollectionView.reloadData()
}
// If we didn't get any data, show the alert view. Have to add a delay because of a weird UI bug.
if assetOverviewDataManager.sensors.count == 0 {
_ = NSTimer.scheduledTimerWithTimeInterval(0.3, target: self, selector: Selector("callPerch"), userInfo: nil, repeats: false)
}
}
func callPerch() {
self.perchAlertManager.displayDefaultPinAlert()
}
override func viewDidDisappear(animated: Bool) {
super.viewDidDisappear(animated)
self.isVisible = false
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
}
// MARK: Worklight calls for asset data
/**
Method to query AssetOverviewDataManager for all asset data
*/
func getAllAssetData() {
let priority = DISPATCH_QUEUE_PRIORITY_DEFAULT
dispatch_async(dispatch_get_global_queue(priority, 0)) {
self.assetOverviewDataManager.getAllAssetData({[unowned self] in self.assetDataReturned($0)})
}
}
/**
Callback method passed into worklight call. Method takes action based on backend query success or failure
- parameter success: Bool representing status of worklight response
*/
func assetDataReturned(success: Bool) {
dispatch_async(dispatch_get_main_queue()) {
if success {
let manager = AssetOverviewDataManager.sharedInstance
if manager.sensors.count != 0 {
self.assetCollectionView.reloadData()
self.perchAlertManager.hideAlertView()
} else {
MQALogger.log("Asset Overview data query was successful, but found no data", withLevel: MQALogLevelWarning)
self.perchAlertManager.displayDefaultPinAlert()
}
} else {
MQALogger.log("Asset Overview data query was unsuccessful", withLevel: MQALogLevelWarning)
self.perchAlertManager.displayDefaultPinAlert()
}
}
}
/**
Method that kicks off assetDetail animation
- parameter assetID: ID for asset to load
*/
func presentViewControllerFromBottom(assetStatus: Int) {
if let del = navDelegate {
del.assetSelected(assetStatus)
}
}
/**
Helper method for reloading the asset overview page to stay in sync with simulator values.
Called when the app comes back into foreground or when a push notification comes in.
*/
class func reload() {
if UIViewController.assetOverviewReference().isKindOfClass(AssetsViewController) {
let assetOverviewVC = UIViewController.assetOverviewReference() as! AssetsViewController
if assetOverviewVC.isVisible {
MQALogger.log("Reloading asset overview because push notification")
AssetOverviewDataManager.sharedInstance.getAllAssetData(assetOverviewVC.assetDataReturned)
} else {
AssetOverviewDataManager.sharedInstance.shouldReload = true
}
} else {
AssetOverviewDataManager.sharedInstance.shouldReload = true
}
}
/**
Method to add a sensor, currently is is not implemented so it throws up an alert
- parameter sender: the sending object
*/
@IBAction func addSensor(sender: AnyObject) {
perchAlertManager.displaySimpleAlertSingleButton(NSLocalizedString("This feature is not implemented.", comment: ""), buttonText: NSLocalizedString("DISMISS", comment: ""), callback: nil)
}
}
extension AssetsViewController: UICollectionViewDataSource, UICollectionViewDelegate, UICollectionViewDelegateFlowLayout {
func collectionView(collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int {
let manager = AssetOverviewDataManager.sharedInstance
if manager.sensors.count > 0 {
return manager.sensors.count
}
return 4
}
func collectionView(collectionView: UICollectionView, cellForItemAtIndexPath indexPath: NSIndexPath) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCellWithReuseIdentifier("assetCell", forIndexPath: indexPath) as! AssetCollectionViewCell
if AssetOverviewDataManager.sharedInstance.sensors.count > 0 {
let sensorData = AssetOverviewDataManager.sharedInstance.sensors[indexPath.row] as SensorData
cell.swapAssetState(sensorData.status)
cell.setIconForDevice(sensorData.deviceType!, sensorStatus: sensorData.status)
cell.typeLabel.text = sensorData.name
} else {
// Enables gray circles to show up as placeholders
cell.swapAssetState(0)
cell.assetIconImageView.image = nil
}
return cell
}
// MARK: CollectionView delegate and layout methods
func collectionView(collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, sizeForItemAtIndexPath indexPath: NSIndexPath) -> CGSize {
var totalCellWidth: CGFloat!
// If on iPad, have tighter spacing
if UIScreen.mainScreen().bounds.size.width >= 768 {
// subtracting 300 so cells are not gigantic on iPad
totalCellWidth = collectionView.frame.size.width - 300
} else {
// Allows cells to increase in size on larger devices
let horizontalSpacing = self.standardEdgeInsets.left * 3
totalCellWidth = collectionView.frame.size.width - horizontalSpacing
}
// Using available cell width, divide by 2 for single cell width and then multiple by aspect ratio for height
let size = CGSizeMake(totalCellWidth/2, (totalCellWidth/2)*1.03)
return size
}
func collectionView(collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, insetForSectionAtIndex section: Int) -> UIEdgeInsets {
// if on iPad, increase collectionview padding essentially
if UIScreen.mainScreen().bounds.size.width >= 768 {
return UIEdgeInsetsMake(8, 110, 0, 110)
}
return self.standardEdgeInsets
}
func collectionView(collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, minimumLineSpacingForSectionAtIndex section: Int) -> CGFloat {
// Without changing the layout a lot, this is a working solution to space items correctly
let bounds = UIScreen.mainScreen().bounds
if bounds.size.height <= 568 {
return 35
} else if bounds.size.height <= 667 {
return 48
} else {
return 64
}
}
func collectionView(collectionView: UICollectionView, didSelectItemAtIndexPath indexPath: NSIndexPath) {
if AssetOverviewDataManager.sharedInstance.sensors.count > 0 {
let sensorData = AssetOverviewDataManager.sharedInstance.sensors[indexPath.row] as SensorData
// If sensor not enabled, display alert explaining that
if !sensorData.enabled {
self.addSensor(self.view)
} else {
// set route for selected asset
CurrentSensorDataManager.sharedInstance.lastSelectedAsset = sensorData.deviceClassId
let specificRoute = ["route":"sensorDetail/\(sensorData.deviceClassId)"]
WL.sharedInstance().sendActionToJS("changePage", withData: specificRoute)
// start off equal so we can detect change later
CurrentSensorDataManager.sharedInstance.prevSensorStatus = sensorData.status
CurrentSensorDataManager.sharedInstance.currentSensorStatus = sensorData.status
// set device ID so we can begin polling in NativeViewController
let appDel = UIApplication.sharedApplication().delegate as! AppDelegate
appDel.hybridViewController.deviceID = sensorData.deviceClassId
self.presentViewControllerFromBottom(sensorData.status)
}
}
}
}
| epl-1.0 | a64102d711bf671221401801dde2e676 | 38.818548 | 194 | 0.652557 | 5.668772 | false | false | false | false |
swifty-iOS/MBNetworkManager | Sample/ViewController.swift | 1 | 2541 | //
// ViewController.swift
// MBNetworkManager
//
// Created by Manish Bhande on 29/12/16.
// Copyright © 2016 Manish Bhande. All rights reserved.
//
import UIKit
class ViewController: UIViewController {
@IBOutlet weak var progressView: UIProgressView!
@IBOutlet weak var label: UILabel!
@IBOutlet weak var activityView: UIActivityIndicatorView!
override func viewDidLoad() {
super.viewDidLoad()
}
@IBAction func downloadTask(_ sender: UIButton) {
self.dwnloadTask()
}
@IBAction func dataTask(_ sender: UIButton) {
self.dataTask()
}
func dataTask() {
self.view.isUserInteractionEnabled = false
self.progressView.progress = 0.0
self.activityView.startAnimating()
self.label.text = "Loading"
let gTask = Task.sampleTask()
MBNetworkManager.shared.add(dataTask: gTask, completion: { (task, error) in
print(error ?? " ")
DispatchQueue.main.async {
self.view.isUserInteractionEnabled = true
self.activityView.stopAnimating()
self.label.text = "\(task.state)"
}
})
gTask.authentication { _ in
return (.useCredential, nil)
}
gTask.trackState { state in
DispatchQueue.main.async {
self.label.text = "\(state)"
}
}
}
func dwnloadTask() {
self.view.isUserInteractionEnabled = false
self.progressView.progress = 0.0
self.activityView.startAnimating()
self.label.text = "Loading"
let dTask = Task.samplePDF()
MBNetworkManager.shared.add(downloadTask: dTask, completion: { (task, error) in
print(error ?? " ")
DispatchQueue.main.async {
self.view.isUserInteractionEnabled = true
self.activityView.stopAnimating()
self.label.text = "\(task.state)"
}
})
dTask.authentication { _ in
return (.useCredential, nil)
}
dTask.trackState { state in
DispatchQueue.main.async {
self.label.text = "\(state)"
}
}
dTask.progress { (per) in
DispatchQueue.main.async {
self.progressView.progress = Float(per/100)
}
print(per)
}
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
}
| mit | 38e04458bb70f13db77aebc6d5fc3d5c | 28.195402 | 87 | 0.574409 | 4.695009 | false | false | false | false |
RCacheaux/BitbucketKit | Carthage/Checkouts/Swinject/Tests/SwinjectStoryboard/OSX/SwinjectStoryboardSpec.swift | 1 | 8958 | //
// SwinjectStoryboardSpec.swift
// Swinject
//
// Created by Yoichi Tagaya on 8/1/15.
// Copyright © 2015 Swinject Contributors. All rights reserved.
//
import Quick
import Nimble
@testable import Swinject
private var swinjectStoryboardSetupCount = 0
extension SwinjectStoryboard {
static func setup() {
swinjectStoryboardSetupCount += 1
}
}
class SwinjectStoryboardSpec: QuickSpec {
override func spec() {
let bundle = NSBundle(forClass: SwinjectStoryboardSpec.self)
var container: Container!
beforeEach {
container = Container()
}
describe("Instantiation from storyboard") {
it("injects view controller dependency definded by initCompleted handler.") {
container.registerForStoryboard(AnimalViewController.self) { r, c in
c.animal = r.resolve(AnimalType.self)
}
container.register(AnimalType.self) { _ in Cat(name: "Mimi") }
let storyboard = SwinjectStoryboard.create(name: "Animals", bundle: bundle, container: container)
let animalViewController = storyboard.instantiateControllerWithIdentifier("AnimalView") as! AnimalViewController
expect(animalViewController.hasAnimal(named: "Mimi")) == true
}
it("injects window controller dependency definded by initCompleted handler.") {
container.registerForStoryboard(AnimalWindowController.self) { r, c in
c.animal = r.resolve(AnimalType.self)
}
container.register(AnimalType.self) { _ in Cat(name: "Mew") }
let storyboard = SwinjectStoryboard.create(name: "Animals", bundle: bundle, container: container)
let animalViewController = storyboard.instantiateControllerWithIdentifier("AnimalWindow") as! AnimalWindowController
expect(animalViewController.hasAnimal(named: "Mew")) == true
}
it("injects dependency to child view controllers.") {
container.registerForStoryboard(AnimalViewController.self) { r, c in
c.animal = r.resolve(AnimalType.self)
}
container.register(AnimalType.self) { _ in Cat() }
.inObjectScope(.Container)
let storyboard = SwinjectStoryboard.create(name: "Tabs", bundle: bundle, container: container)
let tabBarController = storyboard.instantiateControllerWithIdentifier("TabBarController")
let animalViewController1 = tabBarController.childViewControllers[0] as! AnimalViewController
let animalViewController2 = tabBarController.childViewControllers[1] as! AnimalViewController
let cat1 = animalViewController1.animal as! Cat
let cat2 = animalViewController2.animal as! Cat
expect(cat1) === cat2
}
context("with a registration name set as a user defined runtime attribute on Interface Builder") {
it("injects view controller dependency definded by initCompleted handler with the registration name.") {
// The registration name "hachi" is set in the storyboard.
container.registerForStoryboard(AnimalViewController.self, name: "hachi") { r, c in
c.animal = r.resolve(AnimalType.self)
}
container.register(AnimalType.self) { _ in Dog(name: "Hachi") }
// This registration should not be resolved.
container.registerForStoryboard(AnimalViewController.self) { _, c in
c.animal = Cat(name: "Mimi")
}
let storyboard = SwinjectStoryboard.create(name: "Animals", bundle: bundle, container: container)
let animalViewController = storyboard.instantiateControllerWithIdentifier("HachiView") as! AnimalViewController
expect(animalViewController.hasAnimal(named: "Hachi")) == true
}
it("injects window controller dependency definded by initCompleted handler with the registration name.") {
// The registration name "hachi" is set in the storyboard.
container.registerForStoryboard(AnimalWindowController.self, name: "pochi") { r, c in
c.animal = r.resolve(AnimalType.self)
}
container.register(AnimalType.self) { _ in Dog(name: "Pochi") }
// This registration should not be resolved.
container.registerForStoryboard(AnimalWindowController.self) { _, c in
c.animal = Cat(name: "Mimi")
}
let storyboard = SwinjectStoryboard.create(name: "Animals", bundle: bundle, container: container)
let animalViewController = storyboard.instantiateControllerWithIdentifier("PochiWindow") as! AnimalWindowController
expect(animalViewController.hasAnimal(named: "Pochi")) == true
}
}
context("with container hierarchy") {
it("injects view controller dependency definded in the parent container.") {
container.registerForStoryboard(AnimalViewController.self) { r, c in
c.animal = r.resolve(AnimalType.self)
}
container.register(AnimalType.self) { _ in Cat(name: "Mimi") }
let childContainer = Container(parent: container)
let storyboard = SwinjectStoryboard.create(name: "Animals", bundle: bundle, container: childContainer)
let animalViewController = storyboard.instantiateControllerWithIdentifier("AnimalView") as! AnimalViewController
expect(animalViewController.hasAnimal(named: "Mimi")) == true
}
}
}
describe("Initial controller") {
it("injects dependency definded by initCompleted handler.") {
container.registerForStoryboard(AnimalWindowController.self) { r, c in
c.animal = r.resolve(AnimalType.self)
}
container.register(AnimalType.self) { _ in Cat(name: "Mew") }
let storyboard = SwinjectStoryboard.create(name: "Animals", bundle: bundle, container: container)
let animalViewController = storyboard.instantiateInitialController() as! AnimalWindowController
expect(animalViewController.hasAnimal(named: "Mew")) == true
}
}
describe("Factory method") {
it("uses the default shared container if no container is passed.") {
SwinjectStoryboard.defaultContainer.registerForStoryboard(AnimalViewController.self) { _, _ in }
let storyboard = SwinjectStoryboard.create(name: "Animals", bundle: bundle)
let animalViewController = storyboard.instantiateControllerWithIdentifier("AnimalView")
expect(animalViewController).notTo(beNil())
}
afterEach {
SwinjectStoryboard.defaultContainer.removeAll()
}
}
describe("Storyboard reference") {
it("inject dependency to the view controller in the referenced storyboard.") {
SwinjectStoryboard.defaultContainer.registerForStoryboard(AnimalViewController.self) { r, c in
c.animal = r.resolve(AnimalType.self)
}
SwinjectStoryboard.defaultContainer.register(AnimalType.self) { _ in Cat(name: "Mimi") }
let storyboard1 = SwinjectStoryboard.create(name: "Storyboard1", bundle: bundle)
let windowController = storyboard1.instantiateInitialController() as! NSWindowController
let viewController1 = windowController.contentViewController as! ViewController1
viewController1.performSegueWithIdentifier("ToStoryboard2", sender: nil)
expect(viewController1.animalViewController?.hasAnimal(named: "Mimi")).toEventually(beTrue())
}
afterEach {
SwinjectStoryboard.defaultContainer.removeAll()
}
}
describe("Setup") {
it("calls setup function only once.") {
_ = SwinjectStoryboard.create(name: "Animals", bundle: bundle)
_ = SwinjectStoryboard.create(name: "Animals", bundle: bundle)
expect(swinjectStoryboardSetupCount) == 1
}
}
}
}
| apache-2.0 | 3c6d721a001311a3c02c82da39c199ae | 53.95092 | 135 | 0.595958 | 5.979306 | false | false | false | false |
HJianBo/Mqtt | Sources/Mqtt/Packet/PubAckPacket.swift | 1 | 799 | //
// PubackPacket.swift
// Mqtt
//
// Created by Heee on 16/2/2.
// Copyright © 2016年 hjianbo.me. All rights reserved.
//
import Foundation
struct PubAckPacket: Packet {
var fixedHeader: FixedHeader
var packetId: UInt16
var varHeader: Array<UInt8> {
return packetId.bytes
}
var payload = [UInt8]()
init(packetId: UInt16) {
fixedHeader = FixedHeader(type: .puback)
self.packetId = packetId
}
}
extension PubAckPacket: InitializeWithResponse {
init(header: FixedHeader, bytes: [UInt8]) {
fixedHeader = header
packetId = UInt16(bytes[0])*256+UInt16(bytes[1])
}
}
extension PubAckPacket {
public var description: String {
return "PubAck(packetId: \(packetId))"
}
}
| mit | 68378a48139b209a9b729b554ee4a259 | 18.414634 | 56 | 0.614322 | 3.569507 | false | false | false | false |
laurentVeliscek/AudioKit | AudioKit/Common/Nodes/Effects/Reverb/Apple Reverb/AKReverb2.swift | 2 | 8124 | //
// AKReverb2.swift
// AudioKit
//
// Created by Aurelius Prochazka, revision history on Github.
// Copyright (c) 2016 Aurelius Prochazka. All rights reserved.
//
import AVFoundation
/// AudioKit version of Apple's Reverb2 Audio Unit
///
/// - Parameters:
/// - input: Input node to process
/// - dryWetMix: Dry Wet Mix (CrossFade) ranges from 0 to (Default: 0.5)
/// - gain: Gain (Decibels) ranges from -20 to 20 (Default: 0)
/// - minDelayTime: Min Delay Time (Secs) ranges from 0.0001 to 1.0 (Default: 0.008)
/// - maxDelayTime: Max Delay Time (Secs) ranges from 0.0001 to 1.0 (Default: 0.050)
/// - decayTimeAt0Hz: Decay Time At0 Hz (Secs) ranges from 0.001 to 20.0 (Default: 1.0)
/// - decayTimeAtNyquist: Decay Time At Nyquist (Secs) ranges from 0.001 to 20.0 (Default: 0.5)
/// - randomizeReflections: Randomize Reflections (Integer) ranges from 1 to 1000 (Default: 1)
///
public class AKReverb2: AKNode, AKToggleable {
private let cd = AudioComponentDescription(
componentType: kAudioUnitType_Effect,
componentSubType: kAudioUnitSubType_Reverb2,
componentManufacturer: kAudioUnitManufacturer_Apple,
componentFlags: 0,
componentFlagsMask: 0)
internal var internalEffect = AVAudioUnitEffect()
internal var internalAU: AudioUnit = nil
private var lastKnownMix: Double = 50
/// Dry Wet Mix (CrossFade) ranges from 0 to 1 (Default: 0.5)
public var dryWetMix: Double = 0.5 {
didSet {
if dryWetMix < 0 {
dryWetMix = 0
}
if dryWetMix > 1 {
dryWetMix = 1
}
AudioUnitSetParameter(
internalAU,
kReverb2Param_DryWetMix,
kAudioUnitScope_Global, 0,
Float(dryWetMix * 100.0), 0)
}
}
/// Gain (Decibels) ranges from -20 to 20 (Default: 0)
public var gain: Double = 0 {
didSet {
if gain < -20 {
gain = -20
}
if gain > 20 {
gain = 20
}
AudioUnitSetParameter(
internalAU,
kReverb2Param_Gain,
kAudioUnitScope_Global, 0,
Float(gain), 0)
}
}
/// Min Delay Time (Secs) ranges from 0.0001 to 1.0 (Default: 0.008)
public var minDelayTime: Double = 0.008 {
didSet {
if minDelayTime < 0.0001 {
minDelayTime = 0.0001
}
if minDelayTime > 1.0 {
minDelayTime = 1.0
}
AudioUnitSetParameter(
internalAU,
kReverb2Param_MinDelayTime,
kAudioUnitScope_Global, 0,
Float(minDelayTime), 0)
}
}
/// Max Delay Time (Secs) ranges from 0.0001 to 1.0 (Default: 0.050)
public var maxDelayTime: Double = 0.050 {
didSet {
if maxDelayTime < 0.0001 {
maxDelayTime = 0.0001
}
if maxDelayTime > 1.0 {
maxDelayTime = 1.0
}
AudioUnitSetParameter(
internalAU,
kReverb2Param_MaxDelayTime,
kAudioUnitScope_Global, 0,
Float(maxDelayTime), 0)
}
}
/// Decay Time At0 Hz (Secs) ranges from 0.001 to 20.0 (Default: 1.0)
public var decayTimeAt0Hz: Double = 1.0 {
didSet {
if decayTimeAt0Hz < 0.001 {
decayTimeAt0Hz = 0.001
}
if decayTimeAt0Hz > 20.0 {
decayTimeAt0Hz = 20.0
}
AudioUnitSetParameter(
internalAU,
kReverb2Param_DecayTimeAt0Hz,
kAudioUnitScope_Global, 0,
Float(decayTimeAt0Hz), 0)
}
}
/// Decay Time At Nyquist (Secs) ranges from 0.001 to 20.0 (Default: 0.5)
public var decayTimeAtNyquist: Double = 0.5 {
didSet {
if decayTimeAtNyquist < 0.001 {
decayTimeAtNyquist = 0.001
}
if decayTimeAtNyquist > 20.0 {
decayTimeAtNyquist = 20.0
}
AudioUnitSetParameter(
internalAU,
kReverb2Param_DecayTimeAtNyquist,
kAudioUnitScope_Global, 0,
Float(decayTimeAtNyquist), 0)
}
}
/// Randomize Reflections (Integer) ranges from 1 to 1000 (Default: 1)
public var randomizeReflections: Double = 1 {
didSet {
if randomizeReflections < 1 {
randomizeReflections = 1
}
if randomizeReflections > 1000 {
randomizeReflections = 1000
}
AudioUnitSetParameter(
internalAU,
kReverb2Param_RandomizeReflections,
kAudioUnitScope_Global, 0,
Float(randomizeReflections), 0)
}
}
/// Tells whether the node is processing (ie. started, playing, or active)
public var isStarted = true
/// Initialize the reverb2 node
///
/// - Parameters:
/// - input: Input node to process
/// - dryWetMix: Dry Wet Mix (CrossFade) ranges from 0 to 1 (Default: 0.5)
/// - gain: Gain (Decibels) ranges from -20 to 20 (Default: 0)
/// - minDelayTime: Min Delay Time (Secs) ranges from 0.0001 to 1.0 (Default: 0.008)
/// - maxDelayTime: Max Delay Time (Secs) ranges from 0.0001 to 1.0 (Default: 0.050)
/// - decayTimeAt0Hz: Decay Time At0 Hz (Secs) ranges from 0.001 to 20.0 (Default: 1.0)
/// - decayTimeAtNyquist: Decay Time At Nyquist (Secs) ranges from 0.001 to 20.0 (Default: 0.5)
/// - randomizeReflections: Randomize Reflections (Integer) ranges from 1 to 1000 (Default: 1)
///
public init(
_ input: AKNode,
dryWetMix: Double = 0.5,
gain: Double = 0,
minDelayTime: Double = 0.008,
maxDelayTime: Double = 0.050,
decayTimeAt0Hz: Double = 1.0,
decayTimeAtNyquist: Double = 0.5,
randomizeReflections: Double = 1) {
self.dryWetMix = dryWetMix
self.gain = gain
self.minDelayTime = minDelayTime
self.maxDelayTime = maxDelayTime
self.decayTimeAt0Hz = decayTimeAt0Hz
self.decayTimeAtNyquist = decayTimeAtNyquist
self.randomizeReflections = randomizeReflections
internalEffect = AVAudioUnitEffect(audioComponentDescription: cd)
super.init()
self.avAudioNode = internalEffect
AudioKit.engine.attachNode(self.avAudioNode)
input.addConnectionPoint(self)
internalAU = internalEffect.audioUnit
AudioUnitSetParameter(internalAU, kReverb2Param_DryWetMix, kAudioUnitScope_Global, 0, Float(dryWetMix * 100.0), 0)
AudioUnitSetParameter(internalAU, kReverb2Param_Gain, kAudioUnitScope_Global, 0, Float(gain), 0)
AudioUnitSetParameter(internalAU, kReverb2Param_MinDelayTime, kAudioUnitScope_Global, 0, Float(minDelayTime), 0)
AudioUnitSetParameter(internalAU, kReverb2Param_MaxDelayTime, kAudioUnitScope_Global, 0, Float(maxDelayTime), 0)
AudioUnitSetParameter(internalAU, kReverb2Param_DecayTimeAt0Hz, kAudioUnitScope_Global, 0, Float(decayTimeAt0Hz), 0)
AudioUnitSetParameter(internalAU, kReverb2Param_DecayTimeAtNyquist, kAudioUnitScope_Global, 0, Float(decayTimeAtNyquist), 0)
AudioUnitSetParameter(internalAU, kReverb2Param_RandomizeReflections, kAudioUnitScope_Global, 0, Float(randomizeReflections), 0)
}
// MARK: - Control
/// Function to start, play, or activate the node, all do the same thing
public func start() {
if isStopped {
dryWetMix = lastKnownMix
isStarted = true
}
}
/// Function to stop or bypass the node, both are equivalent
public func stop() {
if isPlaying {
lastKnownMix = dryWetMix
dryWetMix = 0
isStarted = false
}
}
}
| mit | 42a0c1acfd80752dd2f668b974ddae61 | 35.267857 | 140 | 0.578656 | 4.346709 | false | false | false | false |
kaylio/ReactiveCocoa | ReactiveCocoa/Swift/Action.swift | 52 | 8322 | /// Represents an action that will do some work when executed with a value of
/// type `Input`, then return zero or more values of type `Output` and/or error
/// out with an error of type `Error`. If no errors should be possible, NoError
/// can be specified for the `Error` parameter.
///
/// Actions enforce serial execution. Any attempt to execute an action multiple
/// times concurrently will return an error.
public final class Action<Input, Output, Error: ErrorType> {
private let executeClosure: Input -> SignalProducer<Output, Error>
private let eventsObserver: Signal<Event<Output, Error>, NoError>.Observer
/// A signal of all events generated from applications of the Action.
///
/// In other words, this will send every `Event` from every signal generated
/// by each SignalProducer returned from apply().
public let events: Signal<Event<Output, Error>, NoError>
/// A signal of all values generated from applications of the Action.
///
/// In other words, this will send every value from every signal generated
/// by each SignalProducer returned from apply().
public let values: Signal<Output, NoError>
/// A signal of all errors generated from applications of the Action.
///
/// In other words, this will send errors from every signal generated by
/// each SignalProducer returned from apply().
public let errors: Signal<Error, NoError>
/// Whether the action is currently executing.
public var executing: PropertyOf<Bool> {
return PropertyOf(_executing)
}
private let _executing: MutableProperty<Bool> = MutableProperty(false)
/// Whether the action is currently enabled.
public var enabled: PropertyOf<Bool> {
return PropertyOf(_enabled)
}
private let _enabled: MutableProperty<Bool> = MutableProperty(false)
/// Whether the instantiator of this action wants it to be enabled.
private let userEnabled: PropertyOf<Bool>
/// Lazy creation and storage of a UI bindable `CocoaAction``. The default behavior
/// force casts the AnyObject? input to match the action's `Input` type. This makes
/// it unsafe for use when the action is paramerterized for something like `Void`
/// input. In those cases, explicitly assign a value to this property that transforms
/// the input to suit your needs.
public lazy var unsafeCocoaAction: CocoaAction = { _ in
CocoaAction(self) { $0 as! Input }
}()
/// This queue is used for read-modify-write operations on the `_executing`
/// property.
private let executingQueue = dispatch_queue_create("org.reactivecocoa.ReactiveCocoa.Action.executingQueue", DISPATCH_QUEUE_SERIAL)
/// Whether the action should be enabled for the given combination of user
/// enabledness and executing status.
private static func shouldBeEnabled(#userEnabled: Bool, executing: Bool) -> Bool {
return userEnabled && !executing
}
/// Initializes an action that will be conditionally enabled, and create a
/// SignalProducer for each input.
public init<P: PropertyType where P.Value == Bool>(enabledIf: P, _ execute: Input -> SignalProducer<Output, Error>) {
executeClosure = execute
userEnabled = PropertyOf(enabledIf)
(events, eventsObserver) = Signal<Event<Output, Error>, NoError>.pipe()
values = events |> map { $0.value } |> ignoreNil
errors = events |> map { $0.error } |> ignoreNil
_enabled <~ enabledIf.producer
|> combineLatestWith(executing.producer)
|> map(Action.shouldBeEnabled)
}
/// Initializes an action that will be enabled by default, and create a
/// SignalProducer for each input.
public convenience init(_ execute: Input -> SignalProducer<Output, Error>) {
self.init(enabledIf: ConstantProperty(true), execute)
}
deinit {
sendCompleted(eventsObserver)
}
/// Creates a SignalProducer that, when started, will execute the action
/// with the given input, then forward the results upon the produced Signal.
///
/// If the action is disabled when the returned SignalProducer is started,
/// the produced signal will send `ActionError.NotEnabled`, and nothing will
/// be sent upon `values` or `errors` for that particular signal.
public func apply(input: Input) -> SignalProducer<Output, ActionError<Error>> {
return SignalProducer { observer, disposable in
var startedExecuting = false
dispatch_sync(self.executingQueue) {
if self._enabled.value {
self._executing.value = true
startedExecuting = true
}
}
if !startedExecuting {
sendError(observer, .NotEnabled)
return
}
self.executeClosure(input).startWithSignal { signal, signalDisposable in
disposable.addDisposable(signalDisposable)
signal.observe(Signal.Observer { event in
observer.put(event.mapError { .ProducerError($0) })
sendNext(self.eventsObserver, event)
})
}
disposable.addDisposable {
self._executing.value = false
}
}
}
}
/// Wraps an Action for use by a GUI control (such as `NSControl` or
/// `UIControl`), with KVO, or with Cocoa Bindings.
public final class CocoaAction: NSObject {
/// The selector that a caller should invoke upon a CocoaAction in order to
/// execute it.
public static let selector: Selector = "execute:"
/// Whether the action is enabled.
///
/// This property will only change on the main thread, and will generate a
/// KVO notification for every change.
public var enabled: Bool {
return _enabled
}
/// Whether the action is executing.
///
/// This property will only change on the main thread, and will generate a
/// KVO notification for every change.
public var executing: Bool {
return _executing
}
private var _enabled = false
private var _executing = false
private let _execute: AnyObject? -> ()
private let disposable = CompositeDisposable()
/// Initializes a Cocoa action that will invoke the given Action by
/// transforming the object given to execute().
public init<Input, Output, Error>(_ action: Action<Input, Output, Error>, _ inputTransform: AnyObject? -> Input) {
_execute = { input in
let producer = action.apply(inputTransform(input))
producer.start()
}
super.init()
disposable += action.enabled.producer
|> observeOn(UIScheduler())
|> start(next: { [weak self] value in
self?.willChangeValueForKey("enabled")
self?._enabled = value
self?.didChangeValueForKey("enabled")
})
disposable += action.executing.producer
|> observeOn(UIScheduler())
|> start(next: { [weak self] value in
self?.willChangeValueForKey("executing")
self?._executing = value
self?.didChangeValueForKey("executing")
})
}
/// Initializes a Cocoa action that will invoke the given Action by
/// always providing the given input.
public convenience init<Input, Output, Error>(_ action: Action<Input, Output, Error>, input: Input) {
self.init(action, { _ in input })
}
deinit {
disposable.dispose()
}
/// Attempts to execute the underlying action with the given input, subject
/// to the behavior described by the initializer that was used.
@IBAction public func execute(input: AnyObject?) {
_execute(input)
}
public override class func automaticallyNotifiesObserversForKey(key: String) -> Bool {
return false
}
}
/// The type of error that can occur from Action.apply, where `E` is the type of
/// error that can be generated by the specific Action instance.
public enum ActionError<E: ErrorType> {
/// The producer returned from apply() was started while the Action was
/// disabled.
case NotEnabled
/// The producer returned from apply() sent the given error.
case ProducerError(E)
}
extension ActionError: ErrorType {
public var nsError: NSError {
switch self {
case .NotEnabled:
return NSError(domain: "org.reactivecocoa.ReactiveCocoa.Action", code: 1, userInfo: [
NSLocalizedDescriptionKey: self.description
])
case let .ProducerError(error):
return error.nsError
}
}
}
extension ActionError: Printable {
public var description: String {
switch self {
case .NotEnabled:
return "Action executed while disabled"
case let .ProducerError(error):
return toString(error)
}
}
}
public func == <E: Equatable>(lhs: ActionError<E>, rhs: ActionError<E>) -> Bool {
switch (lhs, rhs) {
case (.NotEnabled, .NotEnabled):
return true
case let (.ProducerError(left), .ProducerError(right)):
return left == right
default:
return false
}
}
| mit | 4b841610b4c7ea20e2e92af0546509c3 | 31.76378 | 131 | 0.721581 | 4.047665 | false | false | false | false |
Ravichandrane/ExSwift | ExSwiftTests/FloatExtensionsTests.swift | 25 | 4021 | //
// FloatExtensionsTests.swift
// ExSwift
//
// Created by pNre on 04/06/14.
// Copyright (c) 2014 pNre. All rights reserved.
//
import Quick
import Nimble
class FloatExtensionsSpec: QuickSpec {
override func spec() {
/**
* Float.abs
*/
it("abs") {
expect(Float(0).abs()) == Float(0)
expect(Float(-1).abs()).to(beCloseTo(1, within: 0.001))
expect(Float(1).abs()).to(beCloseTo(1, within: 0.001))
expect(Float(-111.2).abs()).to(beCloseTo(111.2, within: 0.001))
expect(Float(111.2).abs()).to(beCloseTo(111.2, within: 0.001))
}
/**
* Float.sqrt
*/
it("sqrt") {
expect(Float(0).sqrt()) == Float(0)
expect(Float(4).sqrt()).to(beCloseTo(2, within: 0.001))
expect(Float(111.2).sqrt()).to(beCloseTo(sqrt(111.2), within: 0.001))
expect(isnan(Float(-10).sqrt())).to(beTrue())
}
/**
* Float.floor
*/
it("floor") {
expect(Float(0).floor()) == Float(0)
expect(Float(4.99999).floor()).to(beCloseTo(4, within: 0.001))
expect(Float(4.001).floor()).to(beCloseTo(4, within: 0.001))
expect(Float(4.5).floor()).to(beCloseTo(4, within: 0.001))
expect(Float(-4.99999).floor()).to(beCloseTo(-5, within: 0.001))
expect(Float(-4.001).floor()).to(beCloseTo(-5, within: 0.001))
expect(Float(-4.5).floor()).to(beCloseTo(-5, within: 0.001))
}
/**
* Float.ceil
*/
it("ceil") {
expect(Float(0).ceil()) == Float(0)
expect(Float(4.99999).ceil()).to(beCloseTo(5, within: 0.001))
expect(Float(4.001).ceil()).to(beCloseTo(5, within: 0.001))
expect(Float(4.5).ceil()).to(beCloseTo(5, within: 0.001))
expect(Float(-4.99999).ceil()).to(beCloseTo(-4, within: 0.001))
expect(Float(-4.001).ceil()).to(beCloseTo(-4, within: 0.001))
expect(Float(-4.5).ceil()).to(beCloseTo(-4, within: 0.001))
}
/**
* Float.round
*/
it("round") {
expect(Float(0).round()) == Float(0)
expect(Float(4.99999999).round()).to(beCloseTo(5, within: 0.001))
expect(Float(4.001).round()).to(beCloseTo(4, within: 0.001))
expect(Float(4.5).round()).to(beCloseTo(5, within: 0.001))
expect(Float(4.3).round()).to(beCloseTo(4, within: 0.001))
expect(Float(4.7).round()).to(beCloseTo(5, within: 0.001))
expect(Float(-4.99999999).round()).to(beCloseTo(-5, within: 0.001))
expect(Float(-4.001).round()).to(beCloseTo(-4, within: 0.001))
expect(Float(-4.5).round()).to(beCloseTo(-5, within: 0.001))
}
/**
* Float.roundToNearest
*/
it("roundToNearest") {
expect(2.5.roundToNearest(0.3)).to(beCloseTo(2.4, within: 0.01))
expect(0.roundToNearest(0.3)).to(beCloseTo(0.0, within: 0.01))
expect(4.0.roundToNearest(2)).to(beCloseTo(4.0, within: 0.01))
expect(10.0.roundToNearest(3)).to(beCloseTo(9.0, within: 0.01))
expect(-2.0.roundToNearest(3)).to(beCloseTo(-3.0, within: 0.01))
}
/**
* Float.clamp
*/
it("clamp") {
expect(Float(0.25).clamp(0, 0.5)).to(beCloseTo(0.25, within: 0.01))
expect(Float(2).clamp(0, 0.5)).to(beCloseTo(0.5, within: 0.01))
expect(Float(-2).clamp(0, 0.5)).to(beCloseTo(0, within: 0.01))
}
}
}
| bsd-2-clause | bbad377ad968d8b460e7e519fab3b176 | 31.168 | 81 | 0.464312 | 3.672146 | false | false | false | false |
jakarmy/swift-summary | The Swift Summary Book.playground/Pages/22 Protocols.xcplaygroundpage/Contents.swift | 1 | 17552 |
// |=------------------------------------------------------=|
// Copyright (c) 2016 Juan Antonio Karmy.
// Licensed under MIT License
//
// See https://developer.apple.com/library/ios/documentation/Swift/Conceptual/Swift_Programming_Language/ for Swift Language Reference
//
// See Juan Antonio Karmy - http://karmy.co | http://twitter.com/jkarmy
//
// |=------------------------------------------------------=|
import UIKit
/*
===============
Protocol Syntax
===============
*/
protocol SomeProtocol {
// protocol definition goes here
}
protocol AnotherProtocol {
// protocol definition goes here
}
class SomeSuperclass {
}
//If a class has a superclass, list the superclass name before any protocols it adopts, followed by a comma.
class SomeClass: SomeSuperclass, SomeProtocol, AnotherProtocol {
// class definition goes here
}
/*
=====================
Property Requirements
=====================
*/
/* If a protocol requires a property to be gettable and settable, that property requirement cannot be
fulfilled by a constant stored property or a read-only computed property. If the protocol only requires a property to be gettable,
the requirement can be satisfied by any kind of property, and it is valid for the property to be also settable if this is useful for your own code.
*/
protocol SomeOtherProtocol {
var mustBeSettable: Int { get set }
var doesNotNeedToBeSettable: Int { get }
}
protocol FullyNamed {
var fullName: String { get }
}
// Here’s an example of a simple structure that adopts and conforms to the FullyNamed protocol.
struct Person: FullyNamed {
var fullName: String
}
let john = Person(fullName: "John Appleseed")
class Starship: FullyNamed {
var prefix: String?
var name: String
init(name: String, prefix: String? = nil) {
self.name = name
self.prefix = prefix
}
// Computed property.
var fullName: String {
return (prefix != nil ? prefix! + " " : "") + name
}
}
var ncc1701 = Starship(name: "Enterprise", prefix: "USS")
/*
===================
Method Requirements
===================
*/
protocol RandomNumberGenerator {
func random() -> Double
}
class LinearCongruentialGenerator: RandomNumberGenerator {
var lastRandom = 42.0
let m = 139_968.0
let a = 3_877.0
let c = 29_573.0
func random() -> Double {
lastRandom = ((lastRandom * a + c) .truncatingRemainder(dividingBy: m))
return lastRandom / m
}
}
var generator = LinearCongruentialGenerator()
print("Here's a random number: \(generator.random())")
// prints "Here's a random number: 0.37464991998171"
print("And another one: \(generator.random())")
// prints "And another one: 0.729023776863283"
/*
============================
Mutating Method Requirements
============================
*/
/*
If you define a protocol instance method requirement that is intended to mutate instances
of any type that adopts the protocol, mark the method with the mutating keyword as part
of the protocol’s definition. This enables structures and enumerations to adopt the protocol and satisfy that method requirement.
NOTE
If you mark a protocol instance method requirement as mutating, you do not need to
write the mutating keyword when writing an implementation of that method for a class.
The mutating keyword is only used by structures and enumerations.
*/
protocol Togglable {
mutating func toggle()
}
enum OnOffSwitch: Togglable {
case Off, On
mutating func toggle() {
switch self {
case Off:
self = On
case On:
self = Off
}
}
}
var lightSwitch = OnOffSwitch.Off
lightSwitch.toggle()
// lightSwitch is now equal to .On
/*
========================
Initializer Requirements
========================
*/
protocol SomeNewProtocol {
init(someParameter: Int)
}
/*
You can implement a protocol initializer requirement on a conforming class as either a designated initializer
or a convenience initializer. In both cases, you must mark the initializer implementation with the required modifier:
*/
class SomeNewClass: SomeNewProtocol {
required init(someParameter: Int) {
// initializer implementation goes here
}
}
/*
NOTE
You do not need to mark protocol initializer implementations with the required modifier on classes
that are marked with the final modifier, because final classes cannot be subclassed. For more on the final modifier, see Preventing Overrides.
*/
class SomeSuperClass {
init() {
// initializer implementation goes here
}
}
class SomeSubClass: SomeSuperClass, SomeProtocol {
// "required" from SomeProtocol conformance; "override" from SomeSuperClass
required override init() {
// initializer implementation goes here
}
}
/*
==================
Protocols as Types
==================
*/
/*
Because it is a type, you can use a protocol in many places where other types are allowed, including:
- As a parameter type or return type in a function, method, or initializer
- As the type of a constant, variable, or property
- As the type of items in an array, dictionary, or other container
NOTE
Because protocols are types, begin their names with a capital letter (such as FullyNamed and RandomNumberGenerator) to match the names of other types in Swift (such as Int, String, and Double).
*/
class Dice {
let sides: Int
let generator: RandomNumberGenerator
init(sides: Int, generator: RandomNumberGenerator) {
self.sides = sides
self.generator = generator
}
func roll() -> Int {
return Int(generator.random() * Double(sides)) + 1
}
}
var d6 = Dice(sides: 6, generator: LinearCongruentialGenerator())
for _ in 1...5 {
print("Random dice roll is \(d6.roll())")
}
// Random dice roll is 3
// Random dice roll is 5
// Random dice roll is 4
// Random dice roll is 5
// Random dice roll is 4
/*
==========
Delegation
==========
*/
protocol DiceGame {
var dice: Dice { get }
func play()
}
protocol DiceGameDelegate {
func gameDidStart(game: DiceGame)
func game(game: DiceGame, didStartNewTurnWithDiceRoll diceRoll: Int)
func gameDidEnd(game: DiceGame)
}
class SnakesAndLadders: DiceGame {
let finalSquare = 25
let dice = Dice(sides: 6, generator: LinearCongruentialGenerator())
var square = 0
var board: [Int]
init() {
board = [Int](repeating: 0, count: finalSquare + 1)
board[03] = +08; board[06] = +11; board[09] = +09; board[10] = +02
board[14] = -10; board[19] = -11; board[22] = -02; board[24] = -08
}
var delegate: DiceGameDelegate?
func play() {
square = 0
delegate?.gameDidStart(game: self)
gameLoop: while square != finalSquare {
let diceRoll = dice.roll()
delegate?.game(game: self, didStartNewTurnWithDiceRoll: diceRoll)
switch square + diceRoll {
case finalSquare:
break gameLoop
case let newSquare where newSquare > finalSquare:
continue gameLoop
default:
square += diceRoll
square += board[square]
}
}
delegate?.gameDidEnd(game: self)
}
}
/*
Note that the delegate property is defined as an optional DiceGameDelegate,
because a delegate isn’t required in order to play the game. Because it is of an optional type,
the delegate property is automatically set to an initial value of nil. Thereafter, the game instantiator has the option to set the property to a suitable delegate.
*/
class DiceGameTracker: DiceGameDelegate {
var numberOfTurns = 0
func gameDidStart(game: DiceGame) {
numberOfTurns = 0
if game is SnakesAndLadders {
print("Started a new game of Snakes and Ladders")
}
print("The game is using a \(game.dice.sides)-sided dice")
}
func game(game: DiceGame, didStartNewTurnWithDiceRoll diceRoll: Int) {
numberOfTurns += 1
print("Rolled a \(diceRoll)")
}
func gameDidEnd(game: DiceGame) {
print("The game lasted for \(numberOfTurns) turns")
}
}
// In action:
let tracker = DiceGameTracker()
let game = SnakesAndLadders()
game.delegate = tracker
game.play()
/*
=============================================
Adding Protocol Conformance with an Extension
=============================================
*/
/*
You can extend an existing type to adopt and conform to a new protocol,
even if you do not have access to the source code for the existing type.
Extensions can add new properties, methods, and subscripts to an existing type,
and are therefore able to add any requirements that a protocol may demand
*/
protocol TextRepresentable {
var textualDescription: String { get }
}
extension Dice: TextRepresentable {
var textualDescription: String {
return "A \(sides)-sided dice"
}
}
let d12 = Dice(sides: 12, generator: LinearCongruentialGenerator())
print(d12.textualDescription)
// prints "A 12-sided dice"
/* Declaring Protocol Adoption with an Extension */
struct Hamster {
var name: String
var textualDescription: String {
return "A hamster named \(name)"
}
}
extension Hamster: TextRepresentable {}
// Protocols can also be added to collections
let simon = Hamster(name: "Simon")
let things: [TextRepresentable] = [d12, simon]
for thing in things {
print(thing.textualDescription)
}
/*
====================
Protocol Inheritance
====================
*/
protocol PrettyTextRepresentable: TextRepresentable {
var prettyTextualDescription: String { get }
}
extension SnakesAndLadders: TextRepresentable {
var textualDescription: String {
return "A game of Snakes and Ladders with \(finalSquare) squares"
}
}
extension SnakesAndLadders: PrettyTextRepresentable {
var prettyTextualDescription: String {
var output = textualDescription + ":\n"
for index in 1...finalSquare {
switch board[index] {
case let ladder where ladder > 0:
output += "▲ "
case let snake where snake < 0:
output += "▼ "
default:
output += "○ "
}
}
return output
}
}
/*
This example defines a new protocol, PrettyTextRepresentable, which inherits from TextRepresentable.
Anything that adopts PrettyTextRepresentable must satisfy all of the requirements enforced by TextRepresentable,
plus the additional requirements enforced by PrettyTextRepresentable.
*/
/*
====================
Class-Only Protocols
====================
*/
/*
You can limit protocol adoption to class types (and not structures or enumerations) by adding the class keyword
to a protocol’s inheritance list. The class keyword must always appear first in a protocol’s inheritance list, before any inherited protocols
*/
protocol SomeInheritedProtocol {}
protocol SomeClassOnlyProtocol: class, SomeInheritedProtocol {
// class-only protocol definition goes here
}
/*
====================
Protocol Composition
====================
*/
/*
It can be useful to require a type to conform to multiple protocols at once.
You can combine multiple protocols into a single requirement with a protocol composition.
Protocol compositions have the form protocol<SomeProtocol, AnotherProtocol>.
You can list as many protocols within the pair of angle brackets (<>) as you need, separated by commas.
*/
protocol Named {
var name: String { get }
}
protocol Aged {
var age: Int { get }
}
struct Personn: Named, Aged {
var name: String
var age: Int
}
func wishHappyBirthday(celebrator: protocol<Named, Aged>) {
print("Happy birthday \(celebrator.name) - you're \(celebrator.age)!")
}
let birthdayPerson = Personn(name: "Malcolm", age: 21)
wishHappyBirthday(celebrator: birthdayPerson)
// prints "Happy birthday Malcolm - you're 21!"
/*
NOTE
Protocol compositions do not define a new, permanent protocol type.
Rather, they define a temporary local protocol that has the combined requirements of all protocols in the composition.
*/
/*
=================================
Checking for Protocol Conformance
=================================
*/
/*
You can use the is and as operators described in Type Casting to check for protocol conformance,
and to cast to a specific protocol. Checking for and casting to a protocol follows exactly the same syntax
as checking for and casting to a type:
• The is operator returns true if an instance conforms to a protocol and returns false if it does not.
• The as? version of the downcast operator returns an optional value of the protocol’s type, and this value is nil if the instance does not conform to that protocol.
• The as! version of the downcast operator forces the downcast to the protocol type and triggers a runtime error if the downcast does not succeed.
*/
/*
==============================
Optional Protocol Requirements
==============================
*/
/*
Optional requirements are prefixed by the optional modifier as part of the protocol’s definition.
When you use a method or property in an optional requirement, its type automatically becomes an optional.
For example, a method of type (Int) -> String becomes ((Int) -> String)?. Note that the entire function type is wrapped in the optional, not method’s the return value.
You check for an implementation of an optional method by writing a question mark after the name of the method when it is called, such as someOptionalMethod?(someArgument).
NOTE
Optional protocol requirements can only be specified if your protocol is marked with the @objc attribute.
This attribute indicates that the protocol should be exposed to Objective-C code and is described in
Using Swift with Cocoa and Objective-C (Swift 2.1). Even if you are not interoperating with Objective-C,
you need to mark your protocols with the @objc attribute if you want to specify optional requirements.
Note also that @objc protocols can be adopted only by classes that inherit from
Objective-C classes or other @objc classes. They can’t be adopted by structures or enumerations.
*/
@objc protocol CounterDataSource {
@objc optional func incrementForCount(count: Int) -> Int
@objc optional var fixedIncrement: Int { get }
}
class Counter {
var count = 0
var dataSource: CounterDataSource?
func increment() {
if let amount = dataSource?.incrementForCount?(count: count) {
count += amount
} else if let amount = dataSource?.fixedIncrement {
count += amount
}
}
}
/*
Because the call to incrementForCount(_:) can fail for either of these two reasons,
the call returns an optional Int value. This is true even though incrementForCount(_:)
is defined as returning a nonoptional Int value in the definition of CounterDataSource.
*/
/*
===================
Protocol Extensions
===================
*/
extension RandomNumberGenerator {
func randomBool() -> Bool {
return random() > 0.5
}
}
// By creating an extension on the protocol, all conforming types automatically gain this method implementation without any additional modification.
generator = LinearCongruentialGenerator()
print("Here's a random number: \(generator.random())")
// prints "Here's a random number: 0.37464991998171"
print("And here's a random Boolean: \(generator.randomBool())")
// prints "And here's a random Boolean: true"
/*
You can use protocol extensions to provide a default implementation to any method or property requirement of that protocol.
If a conforming type provides its own implementation of a required method or property,
that implementation will be used instead of the one provided by the extension.
NOTE
Protocol requirements with default implementations provided by extensions are distinct from optional protocol requirements.
Although conforming types don’t have to provide their own implementation of either,
requirements with default implementations can be called without optional chaining.
*/
/*
When you define a protocol extension, you can specify constraints that conforming types must satisfy
before the methods and properties of the extension are available. You write these constraints
after the name of the protocol you’re extending using a where clause
*/
extension Collection where Iterator.Element: TextRepresentable {
var textualDescription: String {
let itemsAsText = self.map { $0.textualDescription }
return "[" + itemsAsText.joined(separator: ", ") + "]"
}
}
let murrayTheHamster = Hamster(name: "Murray")
let morganTheHamster = Hamster(name: "Morgan")
let mauriceTheHamster = Hamster(name: "Maurice")
let hamsters = [murrayTheHamster, morganTheHamster, mauriceTheHamster]
// Because Array conforms to CollectionType and the array’s elements conform to the TextRepresentable protocol,
// the array can use the textualDescription property to get a textual representation of its contents.
print(hamsters.textualDescription)
// prints "[A hamster named Murray, A hamster named Morgan, A hamster named Maurice]"
/*
NOTE
If a conforming type satisfies the requirements for multiple constrained extensions that provide implementations
for the same method or property, Swift will use the implementation corresponding to the most specialized constraints.
*/
| mit | 211377e41b35e784112d327f7ade76bb | 29.409722 | 194 | 0.680863 | 4.637543 | false | false | false | false |
mcabasheer/table-cell-progress-bar | TableDownloadProgress/TableDownloadProgress/MasterViewController.swift | 1 | 7336 | //
// MasterViewController.swift
// TableDownloadProgress
//
// Created by Basheer on 23/8/17.
// Copyright © 2017 Basheer. All rights reserved.
//
import UIKit
enum DownloadStatus {
case none
case inProgress
case completed
case failed
}
struct item {
var title : String!
let link = "https://www.videvo.net/?page_id=123&desc=OldFashionedFilmLeaderCountdownVidevo.mov&vid=1351"
var downloadStatus : DownloadStatus = .none
init(title: String) {
self.title = title
}
}
class MasterViewController: UITableViewController {
typealias ProgressHandler = (Int, Float) -> ()
var detailViewController: DetailViewController? = nil
var objects = [Any]()
var items = [item]()
var tableId = 100
var onProgress : ProgressHandler?
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
//navigationItem.leftBarButtonItem = editButtonItem
let addButton = UIBarButtonItem(barButtonSystemItem: .add, target: self, action: #selector(insertNewObject(_:)))
navigationItem.rightBarButtonItem = addButton
// if let split = splitViewController {
// let controllers = split.viewControllers
// detailViewController = (controllers[controllers.count-1] as! UINavigationController).topViewController as? DetailViewController
// }
items.append(item(title: "Video 1"))
items.append(item(title: "Video 2"))
items.append(item(title: "Video 3"))
items.append(item(title: "Video 4"))
self.tableView.rowHeight = 100.0
// self.onProgress = DownloadManager.shared.onProgress
let appDelegate = UIApplication.shared.delegate as! AppDelegate
appDelegate.masterVC = self
self.tableView.tag = 1000
}
override func viewWillAppear(_ animated: Bool) {
clearsSelectionOnViewWillAppear = splitViewController!.isCollapsed
super.viewWillAppear(animated)
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
@objc
func insertNewObject(_ sender: Any) {
objects.insert(NSDate(), at: 0)
let indexPath = IndexPath(row: 0, section: 0)
tableView.insertRows(at: [indexPath], with: .automatic)
}
// MARK: - Segues
override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
if segue.identifier == "showDetail" {
if let indexPath = tableView.indexPathForSelectedRow {
let object = objects[indexPath.row] as! NSDate
let controller = (segue.destination as! UINavigationController).topViewController as! DetailViewController
controller.detailItem = object
controller.navigationItem.leftBarButtonItem = splitViewController?.displayModeButtonItem
controller.navigationItem.leftItemsSupplementBackButton = true
}
}
}
// MARK: - Table View
override func numberOfSections(in tableView: UITableView) -> Int {
return 1
}
override func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return items.count
}
override func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "Cell", for: indexPath)
let item = items[indexPath.row]
cell.textLabel?.text = item.title
if item.downloadStatus != .completed {
let progressRing = UICircularProgressRingView(frame: CGRect(x: 0, y: 0, width: 80, height: 80))
// Change any of the properties you'd like
progressRing.maxValue = 100
progressRing.innerRingColor = UIColor.blue
cell.tag = indexPath.row
cell.accessoryType = UITableViewCellAccessoryType.none
cell.accessoryView = progressRing
}
else {
cell.accessoryView = nil
}
return cell
}
override func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
self.tableView.deselectRow(at: indexPath, animated: true)
var item = items[indexPath.row]
if item.downloadStatus == .inProgress || item.downloadStatus == .completed {
print("video already downloaded")
}
else {
let url = URL(string: item.link)!
let downloadManager = DownloadManager.shared
downloadManager.identifier = indexPath.row
downloadManager.tableId = 1200
downloadManager.folderPath = "video"
let downloadTaskLocal = downloadManager.activate().downloadTask(with: url)
downloadTaskLocal.resume()
downloadManager.onProgress = { (row, tableId, progress) in
//print("Downloading for \(row) with progress \(progress)")
DispatchQueue.main.async {
let appDelegate = UIApplication.shared.delegate as! AppDelegate
if appDelegate.masterVC == nil {
print("master vc is nil")
return
}
if appDelegate.masterVC.tableId != tableId {
// different table
return
}
let indexpath = IndexPath.init(row: row, section: 0)
let cell = appDelegate.masterVC.tableView.cellForRow(at: indexpath)
print("downloading for cell \(String(describing: cell?.tag))")
if progress <= 1.0 {
let progressRing = cell?.accessoryView as! UICircularProgressRingView
progressRing.setProgress(value: CGFloat(progress * 100), animationDuration: 0.2)
if progress == 1.0 {
item.downloadStatus = .completed
cell?.textLabel?.text = "Download Complete"
}
else {
cell?.textLabel?.text = "Download In Progress"
}
}
}
}
}
}
override func tableView(_ tableView: UITableView, canEditRowAt indexPath: IndexPath) -> Bool {
// Return false if you do not want the specified item to be editable.
return true
}
override func tableView(_ tableView: UITableView, commit editingStyle: UITableViewCellEditingStyle, forRowAt indexPath: IndexPath) {
if editingStyle == .delete {
objects.remove(at: indexPath.row)
tableView.deleteRows(at: [indexPath], with: .fade)
} else if editingStyle == .insert {
// Create a new instance of the appropriate class, insert it into the array, and add a new row to the table view.
}
}
}
| gpl-3.0 | 70e18f42540fe24f37caf8be7c202beb | 35.492537 | 141 | 0.586639 | 5.552612 | false | false | false | false |
litoarias/SwiftTemplate | TemplateSwift3/Utils/IBDesignables/RoundedTextField.swift | 1 | 1477 | //
// RoundedTextField.swift
// TemplateSwift3
//
// Created by Hipolito Arias on 13/07/2017.
// Copyright © 2017 Hipolito Arias. All rights reserved.
//
import UIKit
@IBDesignable
class RoundedTextField: UITextField {
var isInvalidTextField = false
@IBInspectable var cornerRadius : CGFloat = 0.0 {
didSet {
layer.cornerRadius = cornerRadius
layer.masksToBounds = cornerRadius > 0
}
}
@IBInspectable var borderWidth: CGFloat = 0 {
didSet {
layer.borderWidth = borderWidth
}
}
@IBInspectable var borderColor: UIColor? {
didSet {
layer.borderColor = borderColor?.cgColor
}
}
override func textRect(forBounds bounds: CGRect) -> CGRect {
return CGRect(x: bounds.origin.x + Constants.Label.padding, y: bounds.origin.y, width: bounds.width, height: bounds.height)
}
override func editingRect(forBounds bounds: CGRect) -> CGRect {
return CGRect(x: bounds.origin.x + Constants.Label.padding, y: bounds.origin.y, width: bounds.width, height: bounds.height)
}
func resetBorderColor() {
layer.borderColor = UIColor.pinkishGrey.cgColor
layer.borderWidth = 0.5
isInvalidTextField = false
}
func highlightBorderColor() {
layer.borderColor = UIColor.tomato.cgColor
layer.borderWidth = 1.0
isInvalidTextField = true
}
}
| mit | 81e82f678365d4e987c9a70dd1f21860 | 25.836364 | 131 | 0.626694 | 4.527607 | false | false | false | false |
shingt/DraggableModalTransition | Example/DraggableModalTransition/ModalViewController.swift | 1 | 5045 | import UIKit
final class ModalViewController: UIViewController {
private struct Constants {
static let verticalOffset: CGFloat = 20
static let cornerRadius: CGFloat = 10
static let sectionHeaderHeight: CGFloat = 52
static let rowHeight: CGFloat = 40
static let numberOfRows: Int = 50
static let cellIdentifier = "Cell"
static let dummyFooterHeight: CGFloat = 200
}
private enum RowType: Int, CaseIterable {
case close
case push
case present
}
private lazy var tableView: UITableView = {
let tableView = UITableView()
tableView.frame = CGRect(x: 0, y: Constants.verticalOffset, width: self.view.bounds.size.width, height: self.view.bounds.size.height)
tableView.backgroundColor = .clear
tableView.tableFooterView = {
let view = UIView(frame: CGRect(x: 0, y: 0, width: tableView.bounds.width, height: Constants.dummyFooterHeight))
view.backgroundColor = .white
return view
}()
tableView.contentInset = UIEdgeInsets(top: 0, left: 0, bottom: -Constants.dummyFooterHeight + Constants.verticalOffset, right: 0)
tableView.scrollIndicatorInsets = UIEdgeInsets(top: tableView.sectionHeaderHeight, left: 0, bottom: Constants.verticalOffset, right: 0)
tableView.layer.cornerRadius = Constants.cornerRadius
tableView.layer.masksToBounds = true
tableView.register(UITableViewCell.self, forCellReuseIdentifier: Constants.cellIdentifier)
tableView.delegate = self
tableView.dataSource = self
return tableView
}()
weak var modalViewControllerDelegate: ModalViewControllerDelegate?
override func viewDidLoad() {
super.viewDidLoad()
view.backgroundColor = .clear
view.addSubview(tableView)
automaticallyAdjustsScrollViewInsets = false
tableView.reloadData()
}
override func viewWillAppear(_ animated: Bool) {
super.viewWillAppear(animated)
navigationController?.navigationBar.isHidden = true
}
override func viewDidAppear(_ animated: Bool) {
super.viewDidAppear(animated)
tableView.flashScrollIndicators()
}
}
extension ModalViewController: UITableViewDelegate {
func tableView(_ tableView: UITableView, heightForHeaderInSection section: Int) -> CGFloat {
return Constants.sectionHeaderHeight
}
func tableView(_ tableView: UITableView, viewForHeaderInSection section: Int) -> UIView? {
let view = UIView(frame: CGRect(x: 0, y: 0, width: tableView.bounds.width, height: Constants.sectionHeaderHeight))
view.backgroundColor = .white
view.roundsTopCorners(radius: Constants.cornerRadius)
let label = UILabel()
label.text = "Awesome Header"
label.sizeToFit()
label.center = view.center
view.addSubview(label)
return view
}
func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
tableView.deselectRow(at: indexPath, animated: true)
let rowType = RowType(rawValue: indexPath.row % RowType.allCases.count)!
switch rowType {
case .close:
dismiss(animated: true, completion: nil)
case .push:
let con = SecondViewController()
navigationController?.pushViewController(con, animated: true)
case .present:
let con = SecondViewController()
present(con, animated: true, completion: nil)
}
}
func scrollViewDidScroll(_ scrollView: UIScrollView) {
modalViewControllerDelegate?.modalViewDidScroll(scrollView)
}
}
extension ModalViewController: UITableViewDataSource {
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return Constants.numberOfRows
}
func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
return Constants.rowHeight
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: Constants.cellIdentifier, for: indexPath)
let rowType = RowType(rawValue: indexPath.row % RowType.allCases.count)!
let text: String = {
switch rowType {
case .close: return "Close"
case .push: return "Push"
case .present: return "Present"
}
}()
cell.textLabel?.text = text
cell.backgroundColor = .white
return cell
}
}
private extension UIView {
func roundsTopCorners(radius: CGFloat) {
let maskPath = UIBezierPath(
roundedRect: bounds,
byRoundingCorners: [.topLeft, .topRight],
cornerRadii: CGSize(width: radius, height: radius)
)
let maskLayer = CAShapeLayer()
maskLayer.frame = bounds
maskLayer.path = maskPath.cgPath
layer.mask = maskLayer
}
}
| mit | 9974b498381140b30c8afb1f004bdc8f | 35.557971 | 143 | 0.664222 | 5.332981 | false | false | false | false |
biohazardlover/ByTrain | ByTrain/CaptchaImageView.swift | 1 | 3009 |
import UIKit
class CaptchaImageView: UIImageView {
private var circleViews: [UIView] = []
var singleTapAction: (() -> Void)?
var doubleTapAction: (() -> Void)?
var captcha: String {
get {
let tappedPoints = NSMutableArray()
for circleView in circleViews {
if let image = image {
tappedPoints.add(Int(circleView.center.x * image.size.width / frame.size.width))
tappedPoints.add(Int(circleView.center.y * image.size.height / frame.size.height))
}
}
return tappedPoints.componentsJoined(by: ",")
}
}
override func awakeFromNib() {
super.awakeFromNib()
let singleTapGestureRecognizer = UITapGestureRecognizer(target: self, action: #selector(CaptchaImageView.singleTapped(_:)))
singleTapGestureRecognizer.numberOfTapsRequired = 1
addGestureRecognizer(singleTapGestureRecognizer)
let doubleTapGestureRecognizer = UITapGestureRecognizer(target: self, action: #selector(CaptchaImageView.doubleTapped(_:)))
doubleTapGestureRecognizer.numberOfTapsRequired = 2
addGestureRecognizer(doubleTapGestureRecognizer)
singleTapGestureRecognizer.require(toFail: doubleTapGestureRecognizer)
}
@objc func singleTapped(_ tapGestureRecognizer: UITapGestureRecognizer) {
if image != nil {
let circleView = UIView(frame: CGRect(x: 0.0, y: 0.0, width: 30.0, height: 30.0))
circleView.backgroundColor = UIColor.clear
circleView.center = tapGestureRecognizer.location(in: self)
circleView.isUserInteractionEnabled = true
circleView.layer.borderColor = UIColor(red: 0.7, green: 0.0, blue: 0.0, alpha: 1.0).cgColor
circleView.layer.borderWidth = 5.0
circleView.layer.cornerRadius = 15.0
let tapGestureRecognizer = UITapGestureRecognizer(target: self, action: #selector(CaptchaImageView.circleViewTapped(_:)))
circleView.addGestureRecognizer(tapGestureRecognizer)
addSubview(circleView)
circleViews.append(circleView)
singleTapAction?()
}
}
@objc func doubleTapped(_ tapGestureRecognizer: UITapGestureRecognizer) {
if image != nil {
doubleTapAction?()
}
}
@objc func circleViewTapped(_ tapGestureRecognizer: UITapGestureRecognizer) {
if let circleView = tapGestureRecognizer.view {
if let index = circleViews.index(of: circleView) {
circleViews.remove(at: index)
}
circleView.removeFromSuperview()
}
singleTapAction?()
}
func clearCircleViews() {
for circleView in circleViews {
circleView.removeFromSuperview()
}
circleViews.removeAll(keepingCapacity: false)
}
}
| mit | fb5e6ae05e4050eb84ec663b8f2d1ce5 | 36.148148 | 133 | 0.619475 | 5.656015 | false | false | false | false |
jstn/IndependentRotation | IndependentRotationWithCamera/IndependentRotationWithCamera/AppDelegate.swift | 1 | 1123 | //
// AppDelegate.swift
// IndependentRotationWithCamera
//
// Created by Justin Ouellette on 3/1/15.
// Copyright (c) 2015 Justin Ouellette. All rights reserved.
//
import UIKit
@UIApplicationMain
class AppDelegate: UIResponder, UIApplicationDelegate {
var fixedWindow: UIWindow!
var rotatingWindow: UIWindow!
func application(application: UIApplication, didFinishLaunchingWithOptions launchOptions: [NSObject : AnyObject]?) -> Bool {
let screenBounds = UIScreen.mainScreen().bounds
let inset: CGFloat = fabs(screenBounds.width - screenBounds.height)
fixedWindow = UIWindow(frame: screenBounds)
fixedWindow.rootViewController = FixedViewController()
fixedWindow.backgroundColor = UIColor.blackColor()
fixedWindow.hidden = false
rotatingWindow = UIWindow(frame: CGRectInset(screenBounds, -inset, -inset))
rotatingWindow.rootViewController = RotatingViewController()
rotatingWindow.backgroundColor = UIColor.clearColor()
rotatingWindow.opaque = false
rotatingWindow.makeKeyAndVisible()
return true
}
}
| mit | 7984b6bcb38293947bac076b3153cb7b | 33.030303 | 128 | 0.724844 | 5.151376 | false | false | false | false |
kgn/KGNAutoLayout | Tests/KGNAutoLayoutTestsFill.swift | 1 | 12118 | //
// KGNAutoLayoutTests.swift
// KGNAutoLayoutTests
//
// Created by David Keegan on 10/12/15.
// Copyright © 2015 David Keegan. All rights reserved.
//
import XCTest
@testable import KGNAutoLayout
class KGNAutoLayoutTestsFill: KGNAutoLayoutTests {
func testFillHorizontally1() {
let priority = UILayoutPriority.required
let view1 = IntrinsicView()
view1.height = 30
let parentView = UIView(frame: self.parentViewFrame)
parentView.addSubview(view1)
parentView.fillHorizontally(withViews: [view1], priority: priority)
parentView.layoutIfNeeded()
var frame1 = CGRect.zero
frame1.size.width = self.parentViewFrame.width
frame1.size.height = view1.height
XCTAssertEqual(view1.frame, frame1)
}
func testFillHorizontally1Seperation() {
let separation: CGFloat = 10
let priority = UILayoutPriority.defaultLow
let view1 = IntrinsicView()
view1.height = 30
let parentView = UIView(frame: self.parentViewFrame)
parentView.addSubview(view1)
parentView.fillHorizontally(withViews: [view1], separation: separation, priority: priority)
parentView.layoutIfNeeded()
var frame1 = CGRect.zero
frame1.origin.x = separation
frame1.size.width = self.parentViewFrame.width-separation*2
frame1.size.height = view1.height
XCTAssertEqual(view1.frame, frame1)
}
func testFillHorizontally2() {
let number: CGFloat = 2
let priority = UILayoutPriority.defaultHigh
let view1 = IntrinsicView()
view1.height = 30
let view2 = IntrinsicView()
view2.height = 10
let parentView = UIView(frame: self.parentViewFrame)
parentView.addSubview(view1)
parentView.addSubview(view2)
parentView.fillHorizontally(withViews: [view1, view2], priority: priority)
parentView.layoutIfNeeded()
var frame1 = CGRect.zero
frame1.size.width = self.parentViewFrame.width/number
frame1.size.height = view1.height
XCTAssertEqual(view1.frame, frame1)
var frame2 = CGRect.zero
frame2.size.width = self.parentViewFrame.width/number
frame2.origin.x = frame2.size.width
frame2.size.height = view2.height
XCTAssertEqual(view2.frame, frame2)
}
func testFillHorizontally2Seperation() {
let number: CGFloat = 2
let separation: CGFloat = 10
let priority = UILayoutPriority.defaultHigh
let view1 = IntrinsicView()
view1.height = 30
let view2 = IntrinsicView()
view2.height = 10
let parentView = UIView(frame: self.parentViewFrame)
parentView.addSubview(view1)
parentView.addSubview(view2)
parentView.fillHorizontally(withViews: [view1, view2], separation: separation, priority: priority)
parentView.layoutIfNeeded()
var frame1 = CGRect.zero
frame1.origin.x = separation
frame1.size.width = (self.parentViewFrame.width-(separation*(number+1)))/number
frame1.size.height = view1.height
XCTAssertEqual(view1.frame, frame1)
var frame2 = CGRect.zero
frame2.size.width = (self.parentViewFrame.width-(separation*(number+1)))/number
frame2.origin.x = frame2.size.width+(separation*number)
frame2.size.height = view2.height
XCTAssertEqual(view2.frame, frame2)
}
func testFillHorizontally3() {
let number: CGFloat = 3
let priority = UILayoutPriority.defaultHigh
let view1 = IntrinsicView()
view1.height = 30
let view2 = IntrinsicView()
view2.height = 10
let view3 = IntrinsicView()
view3.height = 20
let parentView = UIView(frame: self.parentViewFrame)
parentView.addSubview(view1)
parentView.addSubview(view2)
parentView.addSubview(view3)
parentView.fillHorizontally(withViews: [view1, view2, view3], priority: priority)
parentView.layoutIfNeeded()
var frame1 = CGRect.zero
frame1.size.width = self.parentViewFrame.width/number
frame1.size.height = view1.height
XCTAssertEqual(view1.frame, frame1)
var frame2 = CGRect.zero
frame2.size.width = self.parentViewFrame.width/number
frame2.origin.x = frame2.size.width
frame2.size.height = view2.height
XCTAssertEqual(view2.frame, frame2)
var frame3 = CGRect.zero
frame3.size.width = self.parentViewFrame.width/number
frame3.origin.x = frame3.size.width*2
frame3.size.height = view3.height
XCTAssertEqual(view3.frame, frame3)
}
func testFillHorizontally3Seperation() {
let number: CGFloat = 3
let separation: CGFloat = 27
let priority = UILayoutPriority.defaultHigh
let view1 = IntrinsicView()
view1.height = 30
let view2 = IntrinsicView()
view2.height = 10
let view3 = IntrinsicView()
view3.height = 20
let parentView = UIView(frame: self.parentViewFrame)
parentView.addSubview(view1)
parentView.addSubview(view2)
parentView.addSubview(view3)
parentView.fillHorizontally(withViews: [view1, view2, view3], separation: separation, priority: priority)
parentView.layoutIfNeeded()
var frame1 = CGRect.zero
frame1.origin.x = separation
frame1.size.width = (self.parentViewFrame.width-(separation*(number+1)))/number
frame1.size.height = view1.height
XCTAssertEqual(view1.frame, frame1)
var frame2 = CGRect.zero
frame2.size.width = (self.parentViewFrame.width-(separation*(number+1)))/number
frame2.origin.x = frame2.size.width+(separation*2)
frame2.size.height = view2.height
XCTAssertEqual(view2.frame, frame2)
var frame3 = CGRect.zero
frame3.size.width = (self.parentViewFrame.width-(separation*(number+1)))/number
frame3.origin.x = (frame3.size.width*2)+(separation*number)
frame3.size.height = view3.height
XCTAssertEqual(view3.frame, frame3)
}
func testFillVertically1() {
let priority = UILayoutPriority.defaultLow
let view1 = IntrinsicView()
view1.width = 30
let parentView = UIView(frame: self.parentViewFrame)
parentView.addSubview(view1)
parentView.fillVertically(withViews: [view1], priority: priority)
parentView.layoutIfNeeded()
var frame1 = CGRect.zero
frame1.size.height = self.parentViewFrame.height
frame1.size.width = view1.width
XCTAssertEqual(view1.frame, frame1)
}
func testFillVertically1Seperation() {
let separation: CGFloat = 10
let priority = UILayoutPriority.defaultLow
let view1 = IntrinsicView()
view1.width = 30
let parentView = UIView(frame: self.parentViewFrame)
parentView.addSubview(view1)
parentView.fillVertically(withViews: [view1], separation: separation, priority: priority)
parentView.layoutIfNeeded()
var frame1 = CGRect.zero
frame1.origin.y = separation
frame1.size.height = self.parentViewFrame.height-separation*2
frame1.size.width = view1.width
XCTAssertEqual(view1.frame, frame1)
}
func testFillVertically2() {
let number: CGFloat = 2
let priority = UILayoutPriority.defaultHigh
let view1 = IntrinsicView()
view1.width = 30
let view2 = IntrinsicView()
view2.width = 10
let parentView = UIView(frame: self.parentViewFrame)
parentView.addSubview(view1)
parentView.addSubview(view2)
parentView.fillVertically(withViews: [view1, view2], priority: priority)
parentView.layoutIfNeeded()
var frame1 = CGRect.zero
frame1.size.height = self.parentViewFrame.height/number
frame1.size.width = view1.width
XCTAssertEqual(view1.frame, frame1)
var frame2 = CGRect.zero
frame2.size.height = self.parentViewFrame.height/number
frame2.origin.y = frame2.size.height
frame2.size.width = view2.width
XCTAssertEqual(view2.frame, frame2)
}
func testFillVertically2Seperation() {
let number: CGFloat = 2
let separation: CGFloat = 10
let priority = UILayoutPriority.defaultHigh
let view1 = IntrinsicView()
view1.width = 30
let view2 = IntrinsicView()
view2.width = 10
let parentView = UIView(frame: self.parentViewFrame)
parentView.addSubview(view1)
parentView.addSubview(view2)
parentView.fillVertically(withViews: [view1, view2], separation: separation, priority: priority)
parentView.layoutIfNeeded()
var frame1 = CGRect.zero
frame1.origin.y = separation
frame1.size.height = (self.parentViewFrame.height-(separation*(number+1)))/number
frame1.size.width = view1.width
XCTAssertEqual(view1.frame, frame1)
var frame2 = CGRect.zero
frame2.size.height = (self.parentViewFrame.height-(separation*(number+1)))/number
frame2.origin.y = frame2.size.height+(separation*number)
frame2.size.width = view2.width
XCTAssertEqual(view2.frame, frame2)
}
func testFillVertically3() {
let number: CGFloat = 3
let priority = UILayoutPriority.defaultHigh
let view1 = IntrinsicView()
view1.width = 30
let view2 = IntrinsicView()
view2.width = 10
let view3 = IntrinsicView()
view3.width = 20
let parentView = UIView(frame: self.parentViewFrame)
parentView.addSubview(view1)
parentView.addSubview(view2)
parentView.addSubview(view3)
parentView.fillVertically(withViews: [view1, view2, view3], priority: priority)
parentView.layoutIfNeeded()
var frame1 = CGRect.zero
frame1.size.height = self.parentViewFrame.height/number
frame1.size.width = view1.width
XCTAssertEqual(view1.frame, frame1)
var frame2 = CGRect.zero
frame2.size.height = self.parentViewFrame.height/number
frame2.origin.y = frame2.size.height
frame2.size.width = view2.width
XCTAssertEqual(view2.frame, frame2)
var frame3 = CGRect.zero
frame3.size.height = self.parentViewFrame.height/number
frame3.origin.y = (frame3.size.height*2)
frame3.size.width = view3.width
XCTAssertEqual(view3.frame, frame3)
}
func testFillVertically3Seperation() {
let number: CGFloat = 3
let separation: CGFloat = 27
let priority = UILayoutPriority.defaultHigh
let view1 = IntrinsicView()
view1.width = 30
let view2 = IntrinsicView()
view2.width = 10
let view3 = IntrinsicView()
view3.width = 20
let parentView = UIView(frame: self.parentViewFrame)
parentView.addSubview(view1)
parentView.addSubview(view2)
parentView.addSubview(view3)
parentView.fillVertically(withViews: [view1, view2, view3], separation: separation, priority: priority)
parentView.layoutIfNeeded()
var frame1 = CGRect.zero
frame1.origin.y = separation
frame1.size.height = (self.parentViewFrame.height-(separation*(number+1)))/number
frame1.size.width = view1.width
XCTAssertEqual(view1.frame, frame1)
var frame2 = CGRect.zero
frame2.size.height = (self.parentViewFrame.height-(separation*(number+1)))/number
frame2.origin.y = frame2.size.height+(separation*2)
frame2.size.width = view2.width
XCTAssertEqual(view2.frame, frame2)
var frame3 = CGRect.zero
frame3.size.height = (self.parentViewFrame.height-(separation*(number+1)))/number
frame3.origin.y = (frame3.size.height*2)+(separation*number)
frame3.size.width = view3.width
XCTAssertEqual(view3.frame, frame3)
}
}
| mit | 56d32da9c113712f2da67e8b465674cc | 31.748649 | 113 | 0.656103 | 4.202914 | false | false | false | false |
LoopKit/LoopKit | LoopKitUI/ViewModels/TherapySettingsViewModel.swift | 1 | 6961 | //
// TherapySettingsViewModel.swift
// LoopKitUI
//
// Created by Rick Pasetto on 7/13/20.
// Copyright © 2020 LoopKit Authors. All rights reserved.
//
import Combine
import LoopKit
import HealthKit
import SwiftUI
public protocol TherapySettingsViewModelDelegate: AnyObject {
func syncBasalRateSchedule(items: [RepeatingScheduleValue<Double>], completion: @escaping (Result<BasalRateSchedule, Error>) -> Void)
func syncDeliveryLimits(deliveryLimits: DeliveryLimits, completion: @escaping (Result<DeliveryLimits, Error>) -> Void)
func saveCompletion(for therapySetting: TherapySetting, therapySettings: TherapySettings)
func pumpSupportedIncrements() -> PumpSupportedIncrements?
}
public class TherapySettingsViewModel: ObservableObject {
@Published public var therapySettings: TherapySettings
private let initialTherapySettings: TherapySettings
let sensitivityOverridesEnabled: Bool
let adultChildInsulinModelSelectionEnabled: Bool
public var prescription: Prescription?
private weak var delegate: TherapySettingsViewModelDelegate?
public init(therapySettings: TherapySettings,
pumpSupportedIncrements: (() -> PumpSupportedIncrements?)? = nil,
sensitivityOverridesEnabled: Bool = false,
adultChildInsulinModelSelectionEnabled: Bool = false,
prescription: Prescription? = nil,
delegate: TherapySettingsViewModelDelegate? = nil) {
self.therapySettings = therapySettings
self.initialTherapySettings = therapySettings
self.sensitivityOverridesEnabled = sensitivityOverridesEnabled
self.adultChildInsulinModelSelectionEnabled = adultChildInsulinModelSelectionEnabled
self.prescription = prescription
self.delegate = delegate
}
var deliveryLimits: DeliveryLimits {
return DeliveryLimits(maximumBasalRate: therapySettings.maximumBasalRatePerHour.map { HKQuantity(unit: .internationalUnitsPerHour, doubleValue: $0) },
maximumBolus: therapySettings.maximumBolus.map { HKQuantity(unit: .internationalUnit(), doubleValue: $0) } )
}
var suspendThreshold: GlucoseThreshold? {
return therapySettings.suspendThreshold
}
var glucoseTargetRangeSchedule: GlucoseRangeSchedule? {
return therapySettings.glucoseTargetRangeSchedule
}
func glucoseTargetRangeSchedule(for glucoseUnit: HKUnit) -> GlucoseRangeSchedule? {
return glucoseTargetRangeSchedule?.schedule(for: glucoseUnit)
}
var correctionRangeOverrides: CorrectionRangeOverrides {
return CorrectionRangeOverrides(preMeal: therapySettings.correctionRangeOverrides?.preMeal,
workout: therapySettings.correctionRangeOverrides?.workout)
}
var correctionRangeScheduleRange: ClosedRange<HKQuantity> {
precondition(therapySettings.glucoseTargetRangeSchedule != nil)
return therapySettings.glucoseTargetRangeSchedule!.scheduleRange()
}
var insulinSensitivitySchedule: InsulinSensitivitySchedule? {
return therapySettings.insulinSensitivitySchedule
}
func insulinSensitivitySchedule(for glucoseUnit: HKUnit) -> InsulinSensitivitySchedule? {
return insulinSensitivitySchedule?.schedule(for: glucoseUnit)
}
/// Reset to initial
public func reset() {
therapySettings = initialTherapySettings
}
}
// MARK: Passing along to the delegate
extension TherapySettingsViewModel {
public var maximumBasalScheduleEntryCount: Int? {
pumpSupportedIncrements()?.maximumBasalScheduleEntryCount
}
public func pumpSupportedIncrements() -> PumpSupportedIncrements? {
return delegate?.pumpSupportedIncrements()
}
public func syncBasalRateSchedule(items: [RepeatingScheduleValue<Double>], completion: @escaping (Result<BasalRateSchedule, Error>) -> Void) {
delegate?.syncBasalRateSchedule(items: items, completion: completion)
}
public func syncDeliveryLimits(deliveryLimits: DeliveryLimits, completion: @escaping (Result<DeliveryLimits, Error>) -> Void) {
delegate?.syncDeliveryLimits(deliveryLimits: deliveryLimits, completion: completion)
}
}
// MARK: Saving
extension TherapySettingsViewModel {
public func saveCorrectionRange(range: GlucoseRangeSchedule) {
therapySettings.glucoseTargetRangeSchedule = range
delegate?.saveCompletion(for: TherapySetting.glucoseTargetRange, therapySettings: therapySettings)
}
public func saveCorrectionRangeOverride(preset: CorrectionRangeOverrides.Preset,
correctionRangeOverrides: CorrectionRangeOverrides) {
therapySettings.correctionRangeOverrides = correctionRangeOverrides
switch preset {
case .preMeal:
delegate?.saveCompletion(for: TherapySetting.preMealCorrectionRangeOverride, therapySettings: therapySettings)
case .workout:
delegate?.saveCompletion(for: TherapySetting.workoutCorrectionRangeOverride, therapySettings: therapySettings)
}
}
public func saveSuspendThreshold(quantity: HKQuantity, withDisplayGlucoseUnit displayGlucoseUnit: HKUnit) {
therapySettings.suspendThreshold = GlucoseThreshold(unit: displayGlucoseUnit, value: quantity.doubleValue(for: displayGlucoseUnit))
delegate?.saveCompletion(for: TherapySetting.suspendThreshold, therapySettings: therapySettings)
}
public func saveBasalRates(basalRates: BasalRateSchedule) {
therapySettings.basalRateSchedule = basalRates
delegate?.saveCompletion(for: TherapySetting.basalRate, therapySettings: therapySettings)
}
public func saveDeliveryLimits(limits: DeliveryLimits) {
therapySettings.maximumBasalRatePerHour = limits.maximumBasalRate?.doubleValue(for: .internationalUnitsPerHour)
therapySettings.maximumBolus = limits.maximumBolus?.doubleValue(for: .internationalUnit())
delegate?.saveCompletion(for: TherapySetting.deliveryLimits, therapySettings: therapySettings)
}
public func saveInsulinModel(insulinModelPreset: ExponentialInsulinModelPreset) {
therapySettings.defaultRapidActingModel = insulinModelPreset
delegate?.saveCompletion(for: TherapySetting.insulinModel, therapySettings: therapySettings)
}
public func saveCarbRatioSchedule(carbRatioSchedule: CarbRatioSchedule) {
therapySettings.carbRatioSchedule = carbRatioSchedule
delegate?.saveCompletion(for: TherapySetting.carbRatio, therapySettings: therapySettings)
}
public func saveInsulinSensitivitySchedule(insulinSensitivitySchedule: InsulinSensitivitySchedule) {
therapySettings.insulinSensitivitySchedule = insulinSensitivitySchedule
delegate?.saveCompletion(for: TherapySetting.insulinSensitivity, therapySettings: therapySettings)
}
}
| mit | 69bc5d1ef6b8e779e21d9d45fe03eb20 | 43.903226 | 158 | 0.75 | 5.337423 | false | false | false | false |
panyam/SwiftIO | SwiftIODemo/main.swift | 1 | 2195 |
#if os(Linux)
import Glibc
srandom(UInt32(clock()))
#endif
import CoreFoundation
import SwiftIO
Log.debug("Testing....")
class EchoHandler : StreamProducer, StreamConsumer
{
var stream : Stream?
private var buffer = UnsafeMutablePointer<UInt8>.alloc(DEFAULT_BUFFER_LENGTH)
private var length = 0
/**
* Called when the connection has been closed.
*/
func connectionClosed()
{
Log.debug("Good bye!")
}
func receivedReadError(error: ErrorType) {
Log.debug("Read Error: \(error)")
}
func receivedWriteError(error: SocketErrorType) {
Log.debug("Write Error: \(error)")
}
/**
* Called by the stream when it is ready to send data.
* Returns the number of bytes of data available.
*/
func writeDataRequested() -> (buffer: BufferType, length: LengthType)?
{
Log.debug("Write data requested...");
return (buffer, length)
}
/**
* Called into indicate numWritten bytes have been written.
*/
func dataWritten(numWritten: LengthType)
{
length -= numWritten
}
/**
* Called by the stream when it can pass data to be processed.
* Returns a buffer (and length) into which at most length number bytes will be filled.
*/
func readDataRequested() -> (buffer: UnsafeMutablePointer<UInt8>, length: LengthType)?
{
return (buffer, DEFAULT_BUFFER_LENGTH)
}
/**
* Called to process data that has been received.
* It is upto the caller of this interface to consume *all* the data
* provided.
*/
func dataReceived(length: LengthType)
{
self.length = length
self.stream?.setReadyToWrite()
}
}
class EchoFactory : StreamHandler {
func handleStream(var stream : Stream)
{
let handler = EchoHandler()
handler.stream = stream
stream.consumer = handler
stream.producer = handler
}
}
var server = CFSocketServer(nil)
server.streamHandler = EchoFactory()
server.start()
while CFRunLoopRunInMode(kCFRunLoopDefaultMode, 5, false) != CFRunLoopRunResult.Finished {
Log.debug("Clocked ticked...")
}
| apache-2.0 | c48b13d3fa1864d597abd64415fe0031 | 23.120879 | 91 | 0.632802 | 4.312377 | false | false | false | false |
dmitrinesterenko/Phony | Phony/FileManager.swift | 1 | 2023 | //
// FileManager.swift
// Phony
//
// Created by Dmitri Nesterenko on 5/3/15.
// Copyright (c) 2015 Dmitri Nesterenko. All rights reserved.
//
import Foundation
class FileManager{
//MARK: Phony specific methods
static func recordingUrl() -> String{
var documents: AnyObject = NSSearchPathForDirectoriesInDomains( NSSearchPathDirectory.DocumentDirectory, NSSearchPathDomainMask.UserDomainMask, true)[0]
var recordingUrl: String = documents.stringByAppendingPathComponent("Recordings")
return recordingUrl
}
static func dictionariesUrl(dictionaryTitle: String) -> String!{
if let url = NSBundle.mainBundle().resourcePath {
return url.stringByAppendingPathComponent("Dictionaries").stringByAppendingPathComponent(dictionaryTitle)
}
Log.exception("Could not find the dictionary in \(dictionaryTitle)")
return nil
}
static func rootUrl() -> String{
return NSBundle.mainBundle().resourcePath!
}
/*static func getFilesInPath(path: String)->Array{
var fm = NSFileManager()
fm.enumeratorAtPath(path)
}*/
static func recordingStoragePath(recordingId: String) -> NSURL{
var fullPath = "\(self.recordingUrl())/\(recordingFileName(recordingId))"
var url = NSURL.fileURLWithPath(fullPath as String)
println("url : \(url)")
return url!
}
static func recordingFileName(Id : String) -> String {
return "\(Id).caf"
}
//MARK: General file system methods
// This is called from AppDelegate init to create if the path does not yet exist
static func createIfDoesNotExist(path: String){
var fm = NSFileManager()
var error: NSError?
fm.createDirectoryAtPath(path, withIntermediateDirectories: true, attributes: nil, error: &error)
if let e = error{
//TODO: This should freak out
println(e.localizedDescription)
}
}
}
| gpl-2.0 | 91f8277cbe07c1652347d95264005d54 | 30.123077 | 161 | 0.653485 | 5.070175 | false | false | false | false |
mohssenfathi/MTLImage | MTLImage/Sources/Core/Context.swift | 1 | 2557 | //
// MTLContext.swift
// Pods
//
// Created by Mohammad Fathi on 3/10/16.
//
//
import UIKit
// Set to true to use compiled shaders
let useMetalib = false
public
class Context: NSObject {
var device: MTLDevice!
var commandQueue: MTLCommandQueue!
var processingSize: CGSize!
var processingQueue: DispatchQueue!
var needsUpdate: Bool = true
let semaphore = DispatchSemaphore(value: 3)
var source: Input?
var output: Output?
private var internalLibrary: MTLLibrary!
var library: MTLLibrary! {
get {
if internalLibrary == nil {
loadLibrary()
}
return internalLibrary
}
}
deinit {
source = nil
output = nil
}
override init() {
super.init()
device = MTLCreateSystemDefaultDevice()
guard MTLImage.isMetalSupported else { return }
loadLibrary()
self.commandQueue = self.device.makeCommandQueue()
self.processingQueue = DispatchQueue(label: "MTLImageProcessQueue")
// DispatchQueue(label: "MTLImageProcessQueue", attributes: DispatchQueueAttributes.concurrent)
refreshCurrentCommandBuffer()
}
func loadLibrary() {
if useMetalib {
internalLibrary = MTLLib.sharedLibrary(device: device)
assert(internalLibrary != nil)
}
else {
if #available(iOS 10.0, *) {
internalLibrary = try? device.makeDefaultLibrary(bundle: Bundle(for: Camera.self))
} else {
// This shouldn't be used in production. If it is, user might need to add an empty shader to parent project
internalLibrary = device.makeDefaultLibrary()
}
}
}
/* Returns the full filter chain not including source and output (only first targets for now)
TODO: Include filters with multiple targets
*/
var filterChain: [MTLObject] {
guard let source = source else {
return []
}
var chain = [MTLObject]()
var object = source.targets.first as? MTLObject
while object != nil {
chain.append(object!)
object = object?.targets.first as? MTLObject
}
return chain
}
var currentCommandBuffer: MTLCommandBuffer!
func refreshCurrentCommandBuffer() {
currentCommandBuffer = commandQueue.makeCommandBuffer()
}
}
| mit | ef9d539cf6fc7c594408b8d43a52f3d0 | 25.091837 | 123 | 0.583105 | 5.124248 | false | false | false | false |
abbeycode/Carthage | Source/CarthageKit/FrameworkExtensions.swift | 1 | 7032 | //
// FrameworkExtensions.swift
// Carthage
//
// Created by Justin Spahr-Summers on 2014-10-31.
// Copyright (c) 2014 Carthage. All rights reserved.
//
import Argo
import Foundation
import Result
import ReactiveCocoa
extension String {
/// Returns a producer that will enumerate each line of the receiver, then
/// complete.
internal var linesProducer: SignalProducer<String, NoError> {
return SignalProducer { observer, disposable in
(self as NSString).enumerateLinesUsingBlock { (line, stop) in
sendNext(observer, line)
if disposable.disposed {
stop.memory = true
}
}
sendCompleted(observer)
}
}
}
/// Merges `rhs` into `lhs` and returns the result.
internal func combineDictionaries<K, V>(lhs: [K: V], rhs: [K: V]) -> [K: V] {
var result = lhs
for (key, value) in rhs {
result.updateValue(value, forKey: key)
}
return result
}
/// Sends each value that occurs on `signal` combined with each value that
/// occurs on `otherSignal` (repeats included).
internal func permuteWith<T, U, E>(otherSignal: Signal<U, E>) -> Signal<T, E> -> Signal<(T, U), E> {
return { signal in
return Signal { observer in
let lock = NSLock()
lock.name = "org.carthage.CarthageKit.permuteWith"
var signalValues: [T] = []
var signalCompleted = false
var otherValues: [U] = []
var otherCompleted = false
let compositeDisposable = CompositeDisposable()
compositeDisposable += signal.observe(next: { value in
lock.lock()
signalValues.append(value)
for otherValue in otherValues {
sendNext(observer, (value, otherValue))
}
lock.unlock()
}, error: { error in
sendError(observer, error)
}, completed: {
lock.lock()
signalCompleted = true
if otherCompleted {
sendCompleted(observer)
}
lock.unlock()
}, interrupted: {
sendInterrupted(observer)
})
compositeDisposable += otherSignal.observe(next: { value in
lock.lock()
otherValues.append(value)
for signalValue in signalValues {
sendNext(observer, (signalValue, value))
}
lock.unlock()
}, error: { error in
sendError(observer, error)
}, completed: {
lock.lock()
otherCompleted = true
if signalCompleted {
sendCompleted(observer)
}
lock.unlock()
}, interrupted: {
sendInterrupted(observer)
})
return compositeDisposable
}
}
}
/// Sends each value that occurs on `producer` combined with each value that
/// occurs on `otherProducer` (repeats included).
internal func permuteWith<T, U, E>(otherProducer: SignalProducer<U, E>)(producer: SignalProducer<T, E>) -> SignalProducer<(T, U), E> {
return producer.lift(permuteWith)(otherProducer)
}
/// Dematerializes the signal, like dematerialize(), but only yields inner Error
/// events if no values were sent.
internal func dematerializeErrorsIfEmpty<T, E>(signal: Signal<Event<T, E>, E>) -> Signal<T, E> {
return Signal { observer in
var receivedValue = false
var receivedError: E? = nil
return signal.observe(next: { event in
switch event {
case let .Next(value):
receivedValue = true
sendNext(observer, value.value)
case let .Error(error):
receivedError = error.value
case .Completed:
sendCompleted(observer)
case .Interrupted:
sendInterrupted(observer)
}
}, error: { error in
sendError(observer, error)
}, completed: {
if !receivedValue {
if let receivedError = receivedError {
sendError(observer, receivedError)
}
}
sendCompleted(observer)
}, interrupted: {
sendInterrupted(observer)
})
}
}
/// Sends all permutations of the values from the input producers, as they arrive.
///
/// If no input producers are given, sends a single empty array then completes.
internal func permutations<T, E>(producers: [SignalProducer<T, E>]) -> SignalProducer<[T], E> {
var combined: SignalProducer<[T], E> = SignalProducer(value: [])
for producer in producers {
combined = combined
|> permuteWith(producer)
|> map { (var array, value) in
array.append(value)
return array
}
}
return combined
}
extension NSScanner {
/// Returns the current line being scanned.
internal var currentLine: NSString {
// Force Foundation types, so we don't have to use Swift's annoying
// string indexing.
let nsString: NSString = string
let scanRange: NSRange = NSMakeRange(scanLocation, 0)
let lineRange: NSRange = nsString.lineRangeForRange(scanRange)
return nsString.substringWithRange(lineRange)
}
}
extension NSURLSession {
/// Returns a producer that will download a file using the given request. The
/// file will be deleted after the producer terminates.
internal func carthage_downloadWithRequest(request: NSURLRequest) -> SignalProducer<(NSURL, NSURLResponse), NSError> {
return SignalProducer { observer, disposable in
let serialDisposable = SerialDisposable()
let handle = disposable.addDisposable(serialDisposable)
let task = self.downloadTaskWithRequest(request) { (URL, response, error) in
// Avoid invoking cancel(), or the download may be deleted.
handle.remove()
if let URL = URL, response = response {
sendNext(observer, (URL, response))
sendCompleted(observer)
} else {
sendError(observer, error)
}
}
serialDisposable.innerDisposable = ActionDisposable {
task.cancel()
}
task.resume()
}
}
}
extension NSURL: Decodable {
public class func decode(json: JSON) -> Decoded<NSURL> {
return String.decode(json).flatMap { URLString in
return .fromOptional(self(string: URLString))
}
}
}
extension NSFileManager {
/// Creates a directory enumerator at the given URL. Sends each URL
/// enumerated, along with the enumerator itself (so it can be introspected
/// and modified as enumeration progresses).
public func carthage_enumeratorAtURL(URL: NSURL, includingPropertiesForKeys keys: [String], options: NSDirectoryEnumerationOptions, catchErrors: Bool = false) -> SignalProducer<(NSDirectoryEnumerator, NSURL), CarthageError> {
return SignalProducer { observer, disposable in
let enumerator = self.enumeratorAtURL(URL, includingPropertiesForKeys: keys, options: options) { (URL, error) in
if catchErrors {
return true
} else {
sendError(observer, CarthageError.ReadFailed(URL, error))
return false
}
}!
while !disposable.disposed {
if let URL = enumerator.nextObject() as? NSURL {
let value = (enumerator, URL)
sendNext(observer, value)
} else {
break
}
}
sendCompleted(observer)
}
}
}
/// Creates a counted set from a sequence. The counted set is represented as a
/// dictionary where the keys are elements from the sequence and values count
/// how many times elements are present in the sequence.
internal func buildCountedSet<S: SequenceType>(sequence: S) -> [S.Generator.Element: Int] {
return reduce(sequence, [:]) { (var set, elem) in
if let count = set[elem] {
set[elem] = count + 1
}
else {
set[elem] = 1
}
return set
}
}
| mit | e7c6a1b46baed69697ff5e969f8c23a4 | 25.636364 | 226 | 0.692406 | 3.819663 | false | false | false | false |
AnRanScheme/magiGlobe | magi/magiGlobe/Pods/CryptoSwift/Sources/CryptoSwift/Collection+Extension.swift | 13 | 2673 | //
// Collection+Extension.swift
// CryptoSwift
//
// Created by Marcin Krzyzanowski on 02/08/16.
// Copyright © 2016 Marcin Krzyzanowski. All rights reserved.
//
extension Collection where Self.Iterator.Element == UInt8, Self.Index == Int {
func toUInt32Array() -> Array<UInt32> {
let count = self.count
var result = Array<UInt32>()
result.reserveCapacity(16)
for idx in stride(from: self.startIndex, to: self.endIndex, by: 4) {
var val: UInt32 = 0
val |= count > 3 ? UInt32(self[idx.advanced(by: 3)]) << 24 : 0
val |= count > 2 ? UInt32(self[idx.advanced(by: 2)]) << 16 : 0
val |= count > 1 ? UInt32(self[idx.advanced(by: 1)]) << 8 : 0
val |= count > 0 ? UInt32(self[idx]) : 0
result.append(val)
}
return result
}
func toUInt64Array() -> Array<UInt64> {
let count = self.count
var result = Array<UInt64>()
result.reserveCapacity(32)
for idx in stride(from: self.startIndex, to: self.endIndex, by: 8) {
var val: UInt64 = 0
val |= count > 7 ? UInt64(self[idx.advanced(by: 7)]) << 56 : 0
val |= count > 6 ? UInt64(self[idx.advanced(by: 6)]) << 48 : 0
val |= count > 5 ? UInt64(self[idx.advanced(by: 5)]) << 40 : 0
val |= count > 4 ? UInt64(self[idx.advanced(by: 4)]) << 32 : 0
val |= count > 3 ? UInt64(self[idx.advanced(by: 3)]) << 24 : 0
val |= count > 2 ? UInt64(self[idx.advanced(by: 2)]) << 16 : 0
val |= count > 1 ? UInt64(self[idx.advanced(by: 1)]) << 8 : 0
val |= count > 0 ? UInt64(self[idx.advanced(by: 0)]) << 0 : 0
result.append(val)
}
return result
}
/// Initialize integer from array of bytes. Caution: may be slow!
@available(*, deprecated: 0.6.0, message: "Dont use it. Too generic to be fast")
func toInteger<T: Integer>() -> T where T: ByteConvertible, T: BitshiftOperationsType {
if self.count == 0 {
return 0
}
let size = MemoryLayout<T>.size
var bytes = self.reversed() // FIXME: check it this is equivalent of Array(...)
if bytes.count < size {
let paddingCount = size - bytes.count
if (paddingCount > 0) {
bytes += Array<UInt8>(repeating: 0, count: paddingCount)
}
}
if size == 1 {
return T(truncatingBitPattern: UInt64(bytes[0]))
}
var result: T = 0
for byte in bytes.reversed() {
result = result << 8 | T(byte)
}
return result
}
}
| mit | 25dfcadfde743973b5abaf1de28029eb | 35.60274 | 91 | 0.534431 | 3.721448 | false | false | false | false |
filipealva/WWDC15-Scholarship | Filipe Alvarenga/View/ProjectWithGitHubTableViewCell.swift | 1 | 1284 | //
// ProjectWithGitHubTableViewCell.swift
// Filipe Alvarenga
//
// Created by Filipe Alvarenga on 25/04/15.
// Copyright (c) 2015 Filipe Alvarenga. All rights reserved.
//
import UIKit
class ProjectWithGitHubTableViewCell: UITableViewCell {
// MARK: - Properties
@IBOutlet weak var projectIcon: UIImageView!
@IBOutlet weak var projectName: UILabel!
@IBOutlet weak var projectDescription: UILabel!
@IBOutlet weak var gitHubButton: UIButton!
@IBOutlet weak var appStoreButton: UIButton!
var project: Project! {
didSet {
configureProject()
}
}
// MARK: - Life Cycle
override func drawRect(rect: CGRect) {
super.drawRect(rect)
projectIcon.layer.borderWidth = 1.0
projectIcon.layer.borderColor = UIColor.groupTableViewBackgroundColor().CGColor
gitHubButton.layer.borderWidth = 0.5
appStoreButton.layer.borderWidth = 0.5
}
override func layoutSubviews() {
super.layoutSubviews()
layoutIfNeeded()
}
// MARK: - Data Binding
func configureProject() {
projectIcon.image = project.image
projectName.text = project.name
projectDescription.text = project.description
}
}
| mit | 4dfc9f94165ef3f7684690f6f6ded4cb | 24.176471 | 87 | 0.648754 | 4.845283 | false | false | false | false |
MenloHacks/ios-app | Menlo Hacks/Pods/Parchment/Parchment/Classes/PagingCellLayoutAttributes.swift | 1 | 703 | import UIKit
/// A custom `UICollectionViewLayoutAttributes` subclass that adds a
/// `progress` property indicating how far the user has scrolled.
open class PagingCellLayoutAttributes: UICollectionViewLayoutAttributes {
open var progress: CGFloat = 0.0
override open func copy(with zone: NSZone? = nil) -> Any {
let copy = super.copy(with: zone) as! PagingCellLayoutAttributes
copy.progress = progress
return copy
}
override open func isEqual(_ object: Any?) -> Bool {
if let rhs = object as? PagingCellLayoutAttributes {
if progress != rhs.progress {
return false
}
return super.isEqual(object)
} else {
return false
}
}
}
| mit | f782baa4e70351d223c0b64f1c6768a9 | 26.038462 | 73 | 0.681366 | 4.594771 | false | false | false | false |
uptech/Constraid | Tests/ConstraidTests/ExpandFromSizeTests.swift | 1 | 2309 | import XCTest
@testable import Constraid
class ExpandFromSizeTests: XCTestCase {
func testExpandFromWidthOf() {
let viewOne = View()
let viewTwo = View()
viewOne.addSubview(viewTwo)
let proxy = viewOne.constraid.expand(fromWidthOf: viewTwo, times: 2.0, offsetBy: 10.0, priority: Constraid.LayoutPriority(rawValue: 500))
let constraints = proxy.constraintCollection
proxy.activate()
let constraint = viewOne.constraints.first!
XCTAssertEqual(constraints, viewOne.constraints)
XCTAssertEqual(constraint.firstItem as! View, viewOne)
XCTAssertEqual(constraint.firstAttribute, LayoutAttribute.width)
XCTAssertEqual(constraint.relation, LayoutRelation.greaterThanOrEqual)
XCTAssertEqual(constraint.secondItem as! View, viewTwo)
XCTAssertEqual(constraint.secondAttribute, LayoutAttribute.width)
XCTAssertEqual(constraint.multiplier, 2.0)
XCTAssertEqual(constraint.constant, 10.0)
XCTAssertEqual(constraint.priority, LayoutPriority(rawValue: LayoutPriority.RawValue(500)))
XCTAssertEqual(viewOne.translatesAutoresizingMaskIntoConstraints, false)
}
func testExpandFromHeightOf() {
let viewOne = View()
let viewTwo = View()
viewOne.addSubview(viewTwo)
let proxy = viewOne.constraid.expand(fromHeightOf: viewTwo, times: 2.0, offsetBy: 10.0, priority: Constraid.LayoutPriority(rawValue: 500))
let constraints = proxy.constraintCollection
proxy.activate()
let constraint = viewOne.constraints.first!
XCTAssertEqual(constraints, viewOne.constraints)
XCTAssertEqual(constraint.firstItem as! View, viewOne)
XCTAssertEqual(constraint.firstAttribute, LayoutAttribute.height)
XCTAssertEqual(constraint.relation, LayoutRelation.greaterThanOrEqual)
XCTAssertEqual(constraint.secondItem as! View, viewTwo)
XCTAssertEqual(constraint.secondAttribute, LayoutAttribute.height)
XCTAssertEqual(constraint.multiplier, 2.0)
XCTAssertEqual(constraint.constant, 10.0)
XCTAssertEqual(constraint.priority, LayoutPriority(rawValue: LayoutPriority.RawValue(500)))
XCTAssertEqual(viewOne.translatesAutoresizingMaskIntoConstraints, false)
}
}
| mit | 510b6d244a9ec7e2b70c06e539e1514e | 45.18 | 146 | 0.730619 | 5.39486 | false | true | false | false |
tripleCC/GanHuoCode | GanHuo/Controllers/Base/TPCViewController.swift | 1 | 6618 | //
// TPCViewController.swift
// WKCC
//
// Created by tripleCC on 15/11/19.
// Copyright © 2015年 tripleCC. All rights reserved.
//
import UIKit
enum TPCNavigationBarType {
case Line
case GradientView
}
class TPCViewController: UIViewController {
var navigationBarFrame: CGRect? {
get {
return self.navigationController?.navigationBar.frame
}
set {
self.navigationController?.navigationBar.frame = newValue!
let navigationBarBackgroundViewY = newValue!.origin.y < 0 ? newValue!.origin.y : 0
self.navigationBarBackgroundView.frame = CGRect(x: 0, y: navigationBarBackgroundViewY, width: newValue!.width, height: TPCNavigationBarHeight + TPCStatusBarHeight)
}
}
var tabBarFrame: CGRect? {
get {
return self.tabBarController?.tabBar.frame
}
set {
self.tabBarController?.tabBar.frame = newValue!
}
}
var navigationBarType: TPCNavigationBarType = .GradientView {
didSet {
switch navigationBarType {
case .Line:
self.bottomLine.hidden = false
self.bottomView.hidden = true
case .GradientView:
self.bottomLine.hidden = true
self.bottomView.hidden = false
}
}
}
private var bottomLine: CALayer!
private var bottomView: TPCGradientView!
lazy var navigationBarBackgroundView: UIView = {
let navigationBarBackgroundView = UIView(frame: CGRect(x: 0, y: 0, width: TPCScreenWidth, height: TPCNavigationBarHeight + TPCStatusBarHeight))
navigationBarBackgroundView.backgroundColor = TPCConfiguration.navigationBarBackgroundColor
return navigationBarBackgroundView
}()
override func viewDidLoad() {
super.viewDidLoad()
navigationItem.backBarButtonItem = UIBarButtonItem(title: "", style: UIBarButtonItemStyle.Done, target: nil, action: nil)
automaticallyAdjustsScrollViewInsets = false
view.addSubview(navigationBarBackgroundView)
setupNavigationBar()
}
private func setupNavigationBar() {
bottomLine = CALayer()
bottomLine.frame = CGRect(x: 0, y: navigationBarBackgroundView.bounds.height - 0.5, width: TPCScreenWidth, height: 0.5)
bottomLine.backgroundColor = UIColor.lightGrayColor().colorWithAlphaComponent(0.2).CGColor
navigationBarBackgroundView.layer.addSublayer(bottomLine)
bottomLine.hidden = true
bottomView = TPCGradientView(frame:CGRect(x: 0, y: TPCNavigationBarHeight + TPCStatusBarHeight, width: TPCScreenWidth, height: TPCConfiguration.technicalTableViewTopBottomMargin * 2))
bottomView.toColor = UIColor.whiteColor().colorWithAlphaComponent(0)
bottomView.fromColor = UIColor.whiteColor()
bottomView.opacity = 1
navigationBarBackgroundView.addSubview(bottomView)
}
deinit {
debugPrint("\(self) 被释放了")
}
override func viewWillAppear(animated: Bool) {
super.viewWillAppear(animated)
view.bringSubviewToFront(navigationBarBackgroundView)
navigationBarFrame!.origin.y = TPCStatusBarHeight
if navigationItem.title != nil {
TPCUMManager.beginLogPageView(navigationItem.title!)
}
}
override func viewWillDisappear(animated: Bool) {
super.viewWillDisappear(animated)
if navigationItem.title != nil {
TPCUMManager.endLogPageView(navigationItem.title!)
}
}
func adjustBarPositionByVelocity(velocity: CGFloat, contentOffsetY: CGFloat, animated: Bool = true) {
guard let _ = view.window else { return }
var descNavY = navigationBarFrame!.origin.y
var descTabY = tabBarFrame?.origin.y ?? 0
if velocity > 1.0 {
debugPrint("隐藏")
descNavY = -TPCNavigationBarHeight
descTabY = TPCScreenHeight
} else if velocity < -1.0 {
debugPrint("显示")
descNavY = TPCStatusBarHeight
descTabY = TPCScreenHeight - TPCTabBarHeight
} else {
if contentOffsetY <= TPCNavigationBarHeight * 2 + TPCStatusBarHeight {
debugPrint("显示")
descNavY = TPCStatusBarHeight
}
}
let adjustAction = { () -> Void in
self.navigationBarFrame!.origin.y = descNavY
if TPCConfiguration.hideTabBarInHomePageWhenScroll {
self.tabBarFrame?.origin.y = descTabY
}
}
if descNavY != navigationBarFrame!.origin.y {
if animated {
UIView.animateWithDuration(0.3, animations: adjustAction)
} else {
adjustAction()
}
}
}
func adjustBarToOriginPosition(animated: Bool = true) {
adjustBarPositionByVelocity(-2.0, contentOffsetY: 0, animated: animated)
}
func adjustBarToHidenPosition(animated: Bool = true) {
adjustBarPositionByVelocity(2.0, contentOffsetY: 0, animated: animated)
}
}
typealias TPCNotificationManager = TPCViewController
extension TPCNotificationManager {
func registerObserverForApplicationDidEnterBackground() {
NSNotificationCenter.defaultCenter().addObserver(self, selector: #selector(TPCViewController.applicationDidEnterBackground(_:)), name: UIApplicationDidEnterBackgroundNotification, object: nil)
}
func registerObserverForApplicationDidEnterForeground() {
NSNotificationCenter.defaultCenter().addObserver(self, selector: #selector(TPCViewController.applicationDidEnterForeground(_:)), name: UIApplicationWillEnterForegroundNotification, object: nil)
}
func registerReloadTableView() {
NSNotificationCenter.defaultCenter().addObserver(self, selector: #selector(TPCViewController.reloadTableView), name: TPCTechnicalReloadDataNotification, object: nil)
}
func postReloadTableView() {
NSNotificationCenter.defaultCenter().postNotificationName(TPCTechnicalReloadDataNotification, object: nil)
}
func reloadTableView() { }
func removeObserver() {
NSNotificationCenter.defaultCenter().removeObserver(self)
}
func applicationDidEnterForeground(notification: NSNotification) {
print(#function, self)
}
func applicationDidEnterBackground(notification: NSNotification) {
print(#function, self)
adjustBarToOriginPosition(false)
}
} | mit | a841ce15862f94f3d77f78d312f7bb81 | 36.908046 | 201 | 0.662168 | 5.357433 | false | false | false | false |
bingoogolapple/SwiftNote-PartOne | ImagePicker/ImagePicker/MainViewController.swift | 1 | 3120 | //
// MainViewController.swift
// ImagePicker
//
// Created by bingoogol on 14/9/15.
// Copyright (c) 2014年 bingoogol. All rights reserved.
//
import UIKit
let kImageFileName = "image.png"
let documents = NSSearchPathForDirectoriesInDomains(NSSearchPathDirectory.DocumentDirectory, NSSearchPathDomainMask.UserDomainMask, true)
class MainViewController: UIViewController,UIImagePickerControllerDelegate,UINavigationControllerDelegate {
weak var button:UIButton!
override func loadView() {
// 1.实例化根视图,视图实例化时默认是透明的
self.view = UIView(frame: UIScreen.mainScreen().applicationFrame)
// 2.创建一个按钮,等下选择图片后,在按钮中显示选择的图片
var button: UIButton = UIButton.buttonWithType(UIButtonType.Custom) as UIButton
button.frame = CGRectMake(60, 130, 200, 200)
button.setTitle("选择图片", forState: UIControlState.Normal)
button.backgroundColor = UIColor.greenColor()
button.addTarget(self, action: Selector("clickButton"), forControlEvents: UIControlEvents.TouchUpInside)
self.view.addSubview(button)
self.button = button
}
override func viewDidLoad() {
var path = "\(documents[0])/\(kImageFileName)"
var image:UIImage? = UIImage(contentsOfFile: path)
if image != nil {
button.setImage(image, forState: UIControlState.Normal)
}
}
func clickButton() {
var imagePicker = UIImagePickerController()
// 1)照片源
// a 照片库【用相机拍摄以及用电脑同步的】
// b 保存的图像【用相机拍摄的】
// c 照相机
imagePicker.sourceType = UIImagePickerControllerSourceType.PhotoLibrary
// 2)是否允许编辑
imagePicker.allowsEditing = true
// 3)设置代理
imagePicker.delegate = self
// 4)显示照片选择控制器,显示modal窗口
self.presentViewController(imagePicker, animated: true, completion: nil)
}
// 照片选择完成的代理方法,照片信息保存在info中
func imagePickerController(picker: UIImagePickerController, didFinishPickingMediaWithInfo info: [NSObject : AnyObject]) {
// 获取编辑后的照片
var image:UIImage = info["\(UIImagePickerControllerEditedImage)"] as UIImage
button.setImage(image, forState: UIControlState.Normal)
// 关闭照片选择器
// 注意:使用照片选择器选择的图片,只是保存在内存中,如果需要再次使用,需要做保存处理
dismissViewControllerAnimated(true, completion: {
// 要保存照片需要NSData做中转
var imageData:NSData = UIImagePNGRepresentation(image)
// 需要找出沙盒路径
// 设置保存文件名称
var path = "\(documents[0])/\(kImageFileName)"
println(path)
// 保存文件
imageData.writeToFile(path, atomically: true)
})
}
} | apache-2.0 | 26c56864f6753da9dd3829d2c31ead7f | 35.202703 | 137 | 0.654593 | 4.665505 | false | false | false | false |
swift-lang/swift-t | stc/tests/851-checkpoint.swift | 2 | 1427 |
// SKIP-THIS-TEST
// Test checkpointing for data structures
import assert;
import math;
import stats;
import blob;
import string;
main {
int a;
float A[];
bag<int> abag;
a, A, abag = arrayf(10, [blob_from_string("hello"),
blob_from_string("world")]);
assertEqual(a, 10 + 6, "a");
assertEqual(A[0], 6.0, "A[0]");
assertEqual(bag_size(abag), 2, "bag_size(abag)");
bag<int> baga[], ibag;
baga[0] = ibag;
baga[1] = ibag;
ibag += 1;
ibag += 2;
ibag += 2;
foreach slist in g(baga) {
trace("slist: " + string_join(slist, ", "));
}
blob B1[] = h([blob_from_string("hello"),
blob_from_string("world")]);
assertEqual(string_from_blob(B1[0]), "hello", "B1[0]");
assertEqual(string_from_blob(B1[1]), "world", "B1[1]");
assertEqual(size(B1), 2, "size(B1)");
}
@checkpoint
(int a, float A[], bag<int> abag) arrayf (int b, blob B[]) {
trace("arrayf executed args: " + fromint(b));
foreach x, i in B {
A[i] = itof(blob_size(x));
abag += blob_size(x);
}
a = b + toInt(sum_float(A)/itof(size(A)));
}
// NOTE: this test sometimes fails since i isn't sorted into
// canonical order
@checkpoint
(bag<string[]> o) g (bag<int> i[]) {
trace("g executed");
o += [fromint(bag_size(i[0]))];
o += ["1", "2", "3"];
o += ["4", "5", "6"];
o += ["7", "8", "9"];
}
@checkpoint
(blob o[]) h (blob i[]) {
trace("h executed");
o = i;
}
| apache-2.0 | d7a9b4f3e76cf3665923062a124d15e7 | 19.985294 | 60 | 0.550105 | 2.657356 | false | false | false | false |
bingoogolapple/SwiftNote-PartOne | 滚动视图优化/滚动视图优化/MainViewController.swift | 1 | 5006 | //
// MainViewController.swift
// 滚动视图优化
//
// Created by bingoogol on 14/9/11.
// Copyright (c) 2014年 bingoogol. All rights reserved.
//
import UIKit
/**
开发思路:
1.使用三个UIImageView来显示scrollView中的内容,当页面变化时,刷新三个UIImageView中的内容可以达到模拟连续滚动的效果
2.准备工作:
1)图像数组
2)图像文件名的数组,把要显示的图像文件记录在数组中,等需要时再临时加载图像
3.开发工作
1)实例化滚动视图
2)创建图像文件名的数组
3)创建图像视图数组
*/
let kImageCount = 5
class MainViewController: UIViewController,UIScrollViewDelegate {
weak var scrollView:UIScrollView!
weak var label:UILabel!
// 保存滚动视图显示内容的图像视图数组,数组中一共有三张图片
var imageViewList = [UIImageView]()
// 图像“文件名”数组,按照要显示的顺序保存图像文件名
var imageNameList:NSMutableArray = NSMutableArray(capacity: kImageCount)
override func loadView() {
// 1.实例化视图
self.view = UIView(frame: UIScreen.mainScreen().bounds)
// 2.实例化滚动视图
var scrollView = UIScrollView(frame: UIScreen.mainScreen().applicationFrame)
self.view.addSubview(scrollView)
// 3.创建图像文件名的数组
for i in 1 ... kImageCount {
imageNameList.addObject("\(i).jpg")
}
// 4.创建图像视图的数组
for i in 0 ... 2 {
// 注意:全屏子控件的大小要与其父控件的大小相一致,这样便于移植
var imageView = UIImageView(frame: scrollView.bounds)
imageViewList.append(imageView)
// 将三张图像视图添加到scrollView上,后续直接设置位置即可
scrollView.addSubview(imageView)
}
// 5.设置滚动视图属性
// 1)允许分页
scrollView.pagingEnabled = true
// 2)关闭弹簧效果
scrollView.bounces = false
// 3)关闭水平滚动条
scrollView.showsHorizontalScrollIndicator = false
// 4)设置滚动区域大小
var width = scrollView.bounds.size.width
var height = scrollView.bounds.size.height
scrollView.contentSize = CGSizeMake(scrollView.bounds.size.width * CGFloat(kImageCount + 2), height)
// 5)设置代理
scrollView.delegate = self
self.scrollView = scrollView
// 6.添加分页的控件
var label = UILabel(frame: CGRectMake(0, 420, 320, 20))
label.textAlignment = NSTextAlignment.Center
label.font = UIFont.systemFontOfSize(15)
label.backgroundColor = UIColor.clearColor()
label.textColor = UIColor.redColor()
self.view.addSubview(label)
self.label = label
changeContentOffset(1)
setScrollContentWithPage(0)
}
func setScrollContentWithPage(page:Int) {
label.text = "\(page + 1)/共\(kImageCount)页"
// 4 0 1 0
// 0 1 2 1
// 2 3 4 3
// 3 4 0 4
var startI = (page + kImageCount - 1) % kImageCount
var next = (page + 1) % kImageCount
println("\(startI),\(page),\(next)")
// 知道对应的图片文件名的数组下标,可以直接设置imageView数组中的图像
var width = scrollView.bounds.size.width
var height = scrollView.bounds.size.height
for i in 0 ... 2 {
var imageName = imageNameList[(startI + i) % kImageCount] as NSString
println("imageName:\(imageName)")
var imageView = imageViewList[i]
imageView.image = UIImage(named: imageName)
// 挪动位置,需要连续设置三张图片的位置
// 因为多了两张缓存的页面,因此page = 0时,数组中的第0张图片应该显示在1的位置
imageView.frame = CGRectMake(CGFloat(page + i) * width, 0, width, height)
println("frame:\(imageView.frame)")
}
}
// 滚动视图减速事件
func scrollViewDidEndDecelerating(scrollView: UIScrollView) {
var pageNo = Int(scrollView.contentOffset.x / scrollView.bounds.size.width)
println("页面滚动停止\(pageNo)")
// 0,1,2,3,4,5,6 pageNo
// 4,0,1,2,3,4,0
var needAdjust = false
if pageNo == 0 {
pageNo = kImageCount
needAdjust = true
} else if (pageNo == kImageCount + 1) {
pageNo = 1
needAdjust = true
}
if(needAdjust) {
changeContentOffset(pageNo)
}
setScrollContentWithPage(pageNo - 1)
}
func changeContentOffset(pageNo:Int) {
scrollView.contentOffset = CGPointMake(CGFloat(pageNo) * scrollView.bounds.size.width, 0)
}
}
| apache-2.0 | 43cd26c68d95807fb2a957aa635c9b0e | 30.787879 | 108 | 0.596044 | 3.962229 | false | false | false | false |
wangjianquan/wjq-weibo | weibo_wjq/Main/VisitorView/BaseViewController.swift | 1 | 1532 | //
// BaseViewController.swift
// weibo_wjq
//
// Created by landixing on 2017/5/23.
// Copyright © 2017年 WJQ. All rights reserved.
//
import UIKit
class BaseViewController: UIViewController {
// MARK:- 懒加载属性
lazy var visitorView : VisitorView = VisitorView.loadVisitorView()
// MARK:- 定义变量
var isLogin = UserAccount.isLogin()
// MARK:- 系统回调函数
override func loadView() {
isLogin ? super.loadView() : setupVisitorView()
}
override func viewDidLoad() {
super.viewDidLoad()
}
}
extension BaseViewController{
fileprivate func setupVisitorView() {
view = visitorView
//注册按钮
visitorView.registerBtn.addTarget(self, action: #selector(BaseViewController.registerBtnClick), for: .touchUpInside)
//登录按钮
visitorView.loginBtn.addTarget(self, action: #selector(BaseViewController.loginBtnClick), for: .touchUpInside)
}
}
//MARK: -- 注册登录事件
extension BaseViewController {
@objc fileprivate func registerBtnClick() {
print("registerBtnClick")
}
//登录
@objc fileprivate func loginBtnClick() {
let oauth = UIStoryboard(name: "OAuth", bundle: nil)
guard let oauthVC = oauth.instantiateInitialViewController()
else { return }
present(oauthVC, animated: true, completion: nil)
}
}
| apache-2.0 | 010f3173b15a1faccb31dbb53df0e9d2 | 19.375 | 124 | 0.603954 | 4.857616 | false | false | false | false |
ahoppen/swift | benchmark/single-source/DictionaryCompactMapValues.swift | 10 | 1696 | //===--- DictionaryCompactMapValues.swift ---------------------------------===//
//
// This source file is part of the Swift.org open source project
//
// Copyright (c) 2014 - 2018 Apple Inc. and the Swift project authors
// Licensed under Apache License v2.0 with Runtime Library Exception
//
// See https://swift.org/LICENSE.txt for license information
// See https://swift.org/CONTRIBUTORS.txt for the list of Swift project authors
//
//===----------------------------------------------------------------------===//
import TestsUtils
let size = 100
let oddNumbers = stride(from: 1, to: size, by: 2)
let smallOddNumMap: [Int: Int?] =
Dictionary(uniqueKeysWithValues: zip(oddNumbers, oddNumbers))
let compactOddNums: [Int: Int] =
Dictionary(uniqueKeysWithValues: zip(oddNumbers, oddNumbers))
let oddStringMap: [Int: String] = Dictionary(uniqueKeysWithValues:
(1...size).lazy.map { ($0, $0 % 2 == 0 ? "dummy" : "\($0)") })
let t: [BenchmarkCategory] = [.validation, .api, .Dictionary]
public let benchmarks = [
BenchmarkInfo(name: "DictionaryCompactMapValuesOfNilValue",
runFunction: compactMapValues, tags: t,
setUpFunction: { blackHole(smallOddNumMap); blackHole(compactOddNums)},
legacyFactor: 50),
BenchmarkInfo(name: "DictionaryCompactMapValuesOfCastValue",
runFunction: compactMapValuesInt, tags: t,
setUpFunction: { blackHole(oddStringMap); blackHole(compactOddNums)},
legacyFactor: 54),
]
func compactMapValues(n: Int) {
for _ in 1...20*n {
check(smallOddNumMap.compactMapValues({$0}) == compactOddNums)
}
}
func compactMapValuesInt(n: Int) {
for _ in 1...20*n {
check(oddStringMap.compactMapValues(Int.init) == compactOddNums)
}
}
| apache-2.0 | aec396a5bb6381c826889f5905d89c26 | 35.085106 | 80 | 0.670991 | 3.971897 | false | false | false | false |
ahoppen/swift | test/Generics/derived_via_concrete_in_protocol.swift | 6 | 1855 | // RUN: %target-typecheck-verify-swift -warn-redundant-requirements
// RUN: %target-swift-frontend -debug-generic-signatures -typecheck %s 2>&1 | %FileCheck %s
protocol P24 {
associatedtype C: P20
}
protocol P20 { }
struct X24<T: P20> : P24 {
typealias C = T
}
protocol P26 {
associatedtype C: X3
}
struct X26<T: X3> : P26 {
typealias C = T
}
class X3 { }
// CHECK-LABEL: .P25a@
// CHECK-NEXT: Requirement signature: <Self where Self.[P25a]A == X24<Self.[P25a]B>, Self.[P25a]B : P20>
// CHECK-NEXT: Canonical requirement signature: <τ_0_0 where τ_0_0.[P25a]A == X24<τ_0_0.[P25a]B>, τ_0_0.[P25a]B : P20>
protocol P25a {
associatedtype A: P24 // expected-warning{{redundant conformance constraint 'X24<Self.B>' : 'P24'}}
associatedtype B: P20 where A == X24<B>
}
// CHECK-LABEL: .P25b@
// CHECK-NEXT: Requirement signature: <Self where Self.[P25b]A == X24<Self.[P25b]B>, Self.[P25b]B : P20>
// CHECK-NEXT: Canonical requirement signature: <τ_0_0 where τ_0_0.[P25b]A == X24<τ_0_0.[P25b]B>, τ_0_0.[P25b]B : P20>
protocol P25b {
associatedtype A
associatedtype B: P20 where A == X24<B>
}
// CHECK-LABEL: .P27a@
// CHECK-NEXT: Requirement signature: <Self where Self.[P27a]A == X26<Self.[P27a]B>, Self.[P27a]B : X3>
// CHECK-NEXT: Canonical requirement signature: <τ_0_0 where τ_0_0.[P27a]A == X26<τ_0_0.[P27a]B>, τ_0_0.[P27a]B : X3>
protocol P27a {
associatedtype A: P26 // expected-warning{{redundant conformance constraint 'X26<Self.B>' : 'P26'}}
associatedtype B: X3 where A == X26<B>
}
// CHECK-LABEL: .P27b@
// CHECK-NEXT: Requirement signature: <Self where Self.[P27b]A == X26<Self.[P27b]B>, Self.[P27b]B : X3>
// CHECK-NEXT: Canonical requirement signature: <τ_0_0 where τ_0_0.[P27b]A == X26<τ_0_0.[P27b]B>, τ_0_0.[P27b]B : X3>
protocol P27b {
associatedtype A
associatedtype B: X3 where A == X26<B>
}
| apache-2.0 | 15b259a89c418592e717b20f671a967a | 32.436364 | 118 | 0.665579 | 2.512295 | false | false | false | false |
lyft/SwiftLint | Tests/SwiftLintFrameworkTests/ObjectLiteralRuleTests.swift | 1 | 3050 | import SwiftLintFramework
import XCTest
class ObjectLiteralRuleTests: XCTestCase {
// MARK: - Instance Properties
private let imageLiteralTriggeringExamples = ["", ".init"].flatMap { (method: String) -> [String] in
["UI", "NS"].flatMap { (prefix: String) -> [String] in
[
"let image = ↓\(prefix)Image\(method)(named: \"foo\")"
]
}
}
private let colorLiteralTriggeringExamples = ["", ".init"].flatMap { (method: String) -> [String] in
["UI", "NS"].flatMap { (prefix: String) -> [String] in
[
"let color = ↓\(prefix)Color\(method)(red: 0.3, green: 0.3, blue: 0.3, alpha: 1)",
"let color = ↓\(prefix)Color\(method)(red: 100 / 255.0, green: 50 / 255.0, blue: 0, alpha: 1)",
"let color = ↓\(prefix)Color\(method)(white: 0.5, alpha: 1)"
]
}
}
private var allTriggeringExamples: [String] {
return imageLiteralTriggeringExamples + colorLiteralTriggeringExamples
}
// MARK: - Test Methods
func testObjectLiteralWithDefaultConfiguration() {
verifyRule(ObjectLiteralRule.description)
}
func testObjectLiteralWithImageLiteral() {
// Verify ObjectLiteral rule for when image_literal is true.
let baseDescription = ObjectLiteralRule.description
let nonTriggeringColorLiteralExamples = colorLiteralTriggeringExamples.map { example in
example.replacingOccurrences(of: "↓", with: "")
}
let nonTriggeringExamples = baseDescription.nonTriggeringExamples + nonTriggeringColorLiteralExamples
let description = baseDescription.with(nonTriggeringExamples: nonTriggeringExamples)
.with(triggeringExamples: imageLiteralTriggeringExamples)
verifyRule(description, ruleConfiguration: ["image_literal": true, "color_literal": false])
}
func testObjectLiteralWithColorLiteral() {
// Verify ObjectLiteral rule for when color_literal is true.
let baseDescription = ObjectLiteralRule.description
let nonTriggeringImageLiteralExamples = imageLiteralTriggeringExamples.map { example in
example.replacingOccurrences(of: "↓", with: "")
}
let nonTriggeringExamples = baseDescription.nonTriggeringExamples + nonTriggeringImageLiteralExamples
let description = baseDescription.with(nonTriggeringExamples: nonTriggeringExamples)
.with(triggeringExamples: colorLiteralTriggeringExamples)
verifyRule(description, ruleConfiguration: ["image_literal": false, "color_literal": true])
}
func testObjectLiteralWithImageAndColorLiteral() {
// Verify ObjectLiteral rule for when image_literal & color_literal are true.
let description = ObjectLiteralRule.description.with(triggeringExamples: allTriggeringExamples)
verifyRule(description, ruleConfiguration: ["image_literal": true, "color_literal": true])
}
}
| mit | 97f756dd4784950d88d50431d924fae6 | 45.030303 | 111 | 0.659645 | 5.32049 | false | true | false | false |
davidbjames/Unilib | Unilib/Sources/Functional.swift | 1 | 3559 | //
// Functional.swift
// C3
//
// Created by David James on 2021-12-07.
// Copyright © 2021 David B James. All rights reserved.
//
import Foundation
/// Cache the result of a potentially expensive operation
/// by capturing the cache in the returned closure, storing
/// it somewhere, and then calling with the required input.
/// To store this, call it with the callback function signature
/// that performs the potentially expensive work (without
/// actually calling the callback), and then assign the resulting
/// closure to some location where it can be re-used by calling
/// the code with the same input type and getting back the same
/// output either as just-computed or already computed/cached.
///
/// The input type must be Hashable for the cache key.
/// See also `memoize()` that takes a custom cache key
/// if the input type cannot/should not be hashable.
///
/// // On the containing object:
/// let distanceCache = memoize(distance(rectangles:))
/// ...
/// // Somewhere that computes something:
/// distanceCache(.init(<input>))
public func memoize<I:Hashable,O>(_ closure:@escaping (I)->O) -> (_ input:I, _ debug:Bool)->O {
var cache = [I:O]()
return { input, debug in
if let cached = cache[input] {
if debug {
ApiDebug()?.message("Memoized cache hit on \"\(input)\".", icon:"♻️").output()
}
return cached
}
let result = closure(input)
cache[input] = result
return result
}
}
/// Cache the result of a potentially expensive operation
/// by capturing the cache in the returned closure, storing
/// it somewhere, and then calling with the required input.
/// To store this, call it with the callback function signature
/// that performs the potentially expensive work (without
/// actually calling the callback), and then assign the resulting
/// closure to some location where it can be re-used by calling
/// the code with the same input type and getting back the same
/// output either as just-computed or already computed/cached.
///
/// This method requires a custom Hashable cache key.
/// See also `memoize()` that does not require a custom cache
/// key if the input type is already Hashable.
///
/// // On the containing object:
/// let distanceCache = memoizeWithKey(CGPoint.self, distance(rectangles:))
/// ...
/// // Somewhere that computes something:
/// distanceCache(.init(<input>), key)
public func memoizeWithKey<I,O,Key:Hashable>(_:Key.Type, _ closure:@escaping (I)->O) -> (_ input:I, _ key:Key, _ debug:Bool)->O {
var cache = [Key:O]()
return { input, key, debug in
if let cached = cache[key] {
if debug {
ApiDebug()?.message("Memoized cache hit on \"\(key)\".", icon:"♻️").output()
}
return cached
}
let result = closure(input)
cache[key] = result
return result
}
}
// TODO document this when you've had a chance to try it.
public func memoizeRecursively<I:Hashable,O>(_ closure:@escaping ((I,Bool)->O,I)->O) -> (_ input:I, _ debug:Bool)->O {
var cache = [I:O]()
var memo:((I,Bool)->O)!
memo = { input, debug in
if let cached = cache[input] {
if debug {
ApiDebug()?.message("Memoized cache hit on \"\(input)\".", icon:"♻️").output()
}
return cached
}
let result = closure(memo, input)
cache[input] = result
return result
}
return memo
}
| mit | d5792a2ff6fa4a34b702116450f5e4bb | 36.326316 | 129 | 0.627468 | 4.13769 | false | false | false | false |
ashfurrow/Swift-Course | Step 1.playground/Contents.swift | 1 | 766 | import Foundation
print("hello world")
let name = "Ash"
let age = 26
print(name)
print("Hello my name is \(name) and I am \(age) years old")
// Talk about type inference
var number = 3
number = 5
// Talk about constants
let numbers = [1,2,3]
let strings = ["Ash", "Samuel", "Marga"]
// Fast enumeration
for string in strings {
print(string)
}
// ".each" based
strings.map({ (string: String) -> Void in
print(string)
})
strings.map{ (string: String) -> Void in
print(string)
}
strings.filter { (string: String) -> Bool in
return string.hasPrefix("A")
}
strings.filter { $0.hasPrefix("A") }
let luckyNumbers = ["Ash": 17, "Samuel": 11, "Marga": 4243]
for (key, value) in luckyNumbers {
print("The lucky number of \(key) is \(value)")
}
| mit | b6e600c0f782aad84cd059b0cd0aebfa | 16.813953 | 59 | 0.642298 | 3.015748 | false | false | false | false |
MaxHasADHD/TraktKit | Common/Models/TraktScrobble.swift | 1 | 935 | //
// TraktScrobble.swift
// TraktKit
//
// Created by Maximilian Litteral on 11/8/20.
// Copyright © 2020 Maximilian Litteral. All rights reserved.
//
import Foundation
public struct TraktScrobble: Encodable {
public let movie: SyncId?
public let episode: SyncId?
/// Progress percentage between 0 and 100.
public let progress: Float
/// Version number of the app.
public let appVersion: String?
/// Build date of the app.
public let appDate: String?
enum CodingKeys: String, CodingKey {
case movie, episode, progress, appVersion = "app_version", appDate = "app_date"
}
public init(movie: SyncId? = nil, episode: SyncId? = nil, progress: Float, appVersion: String? = nil, appDate: String? = nil) {
self.movie = movie
self.episode = episode
self.progress = progress
self.appVersion = appVersion
self.appDate = appDate
}
}
| mit | a65752a5781aefcb7d2a451bea3959c5 | 28.1875 | 131 | 0.650964 | 4.06087 | false | false | false | false |
ForrestAlfred/firefox-ios | Client/Frontend/Browser/TabManager.swift | 4 | 17940 | /* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/. */
import Foundation
import WebKit
import Storage
import Shared
protocol TabManagerDelegate: class {
func tabManager(tabManager: TabManager, didSelectedTabChange selected: Browser?, previous: Browser?)
func tabManager(tabManager: TabManager, didCreateTab tab: Browser, restoring: Bool)
func tabManager(tabManager: TabManager, didAddTab tab: Browser, atIndex: Int, restoring: Bool)
func tabManager(tabManager: TabManager, didRemoveTab tab: Browser, atIndex index: Int)
func tabManagerDidRestoreTabs(tabManager: TabManager)
func tabManagerDidAddTabs(tabManager: TabManager)
}
// We can't use a WeakList here because this is a protocol.
class WeakTabManagerDelegate {
weak var value : TabManagerDelegate?
init (value: TabManagerDelegate) {
self.value = value
}
func get() -> TabManagerDelegate? {
return value
}
}
// TabManager must extend NSObjectProtocol in order to implement WKNavigationDelegate
class TabManager : NSObject {
private var delegates = [WeakTabManagerDelegate]()
func addDelegate(delegate: TabManagerDelegate) {
assert(NSThread.isMainThread())
delegates.append(WeakTabManagerDelegate(value: delegate))
}
func removeDelegate(delegate: TabManagerDelegate) {
assert(NSThread.isMainThread())
for var i = 0; i < delegates.count; i++ {
var del = delegates[i]
if delegate === del.get() {
delegates.removeAtIndex(i)
return
}
}
}
private var tabs: [Browser] = []
private var _selectedIndex = -1
var selectedIndex: Int { return _selectedIndex }
private let defaultNewTabRequest: NSURLRequest
private let navDelegate: TabManagerNavDelegate
private var configuration: WKWebViewConfiguration
unowned let profile: Profile
init(defaultNewTabRequest: NSURLRequest, profile: Profile) {
self.profile = profile
// Create a common webview configuration with a shared process pool.
configuration = WKWebViewConfiguration()
configuration.processPool = WKProcessPool()
configuration.preferences.javaScriptCanOpenWindowsAutomatically = !(self.profile.prefs.boolForKey("blockPopups") ?? true)
self.defaultNewTabRequest = defaultNewTabRequest
self.navDelegate = TabManagerNavDelegate()
super.init()
addNavigationDelegate(self)
NSNotificationCenter.defaultCenter().addObserver(self, selector: "prefsDidChange", name: NSUserDefaultsDidChangeNotification, object: nil)
}
deinit {
NSNotificationCenter.defaultCenter().removeObserver(self)
}
func addNavigationDelegate(delegate: WKNavigationDelegate) {
self.navDelegate.insert(delegate)
}
var count: Int {
return tabs.count
}
var selectedTab: Browser? {
if !(0..<count ~= _selectedIndex) {
return nil
}
return tabs[_selectedIndex]
}
subscript(index: Int) -> Browser? {
if index >= tabs.count {
return nil
}
return tabs[index]
}
subscript(webView: WKWebView) -> Browser? {
for tab in tabs {
if tab.webView === webView {
return tab
}
}
return nil
}
func selectTab(tab: Browser?) {
assert(NSThread.isMainThread())
if selectedTab === tab {
return
}
let previous = selectedTab
_selectedIndex = -1
for i in 0..<count {
if tabs[i] === tab {
_selectedIndex = i
break
}
}
assert(tab === selectedTab, "Expected tab is selected")
selectedTab?.createWebview()
for delegate in delegates {
delegate.get()?.tabManager(self, didSelectedTabChange: tab, previous: previous)
}
}
// This method is duplicated to hide the flushToDisk option from consumers.
func addTab(var request: NSURLRequest! = nil, configuration: WKWebViewConfiguration! = nil) -> Browser {
return self.addTab(request: request, configuration: configuration, flushToDisk: true, zombie: false)
}
func addTabsForURLs(urls: [NSURL], zombie: Bool) {
if urls.isEmpty {
return
}
var tab: Browser!
for (let url) in urls {
tab = self.addTab(request: NSURLRequest(URL: url), flushToDisk: false, zombie: zombie, restoring: true)
}
// Flush.
storeChanges()
// Select the most recent.
self.selectTab(tab)
// Notify that we bulk-loaded so we can adjust counts.
for delegate in delegates {
delegate.get()?.tabManagerDidAddTabs(self)
}
}
func addTab(var request: NSURLRequest! = nil, configuration: WKWebViewConfiguration! = nil, flushToDisk: Bool, zombie: Bool, restoring: Bool = false) -> Browser {
assert(NSThread.isMainThread())
configuration?.preferences.javaScriptCanOpenWindowsAutomatically = !(self.profile.prefs.boolForKey("blockPopups") ?? true)
let tab = Browser(configuration: configuration ?? self.configuration)
for delegate in delegates {
delegate.get()?.tabManager(self, didCreateTab: tab, restoring: restoring)
}
tabs.append(tab)
for delegate in delegates {
delegate.get()?.tabManager(self, didAddTab: tab, atIndex: tabs.count - 1, restoring: restoring)
}
if !zombie {
tab.createWebview()
}
tab.navigationDelegate = self.navDelegate
tab.loadRequest(request ?? defaultNewTabRequest)
if flushToDisk {
storeChanges()
}
return tab
}
// This method is duplicated to hide the flushToDisk option from consumers.
func removeTab(tab: Browser) {
self.removeTab(tab, flushToDisk: true)
hideNetworkActivitySpinner()
}
private func removeTab(tab: Browser, flushToDisk: Bool) {
assert(NSThread.isMainThread())
// If the removed tab was selected, find the new tab to select.
if tab === selectedTab {
let index = getIndex(tab)
if index + 1 < count {
selectTab(tabs[index + 1])
} else if index - 1 >= 0 {
selectTab(tabs[index - 1])
} else {
assert(count == 1, "Removing last tab")
selectTab(nil)
}
}
let prevCount = count
var index = -1
for i in 0..<count {
if tabs[i] === tab {
tabs.removeAtIndex(i)
index = i
break
}
}
assert(count == prevCount - 1, "Tab removed")
// There's still some time between this and the webView being destroyed.
// We don't want to pick up any stray events.
tab.webView?.navigationDelegate = nil
for delegate in delegates {
delegate.get()?.tabManager(self, didRemoveTab: tab, atIndex: index)
}
if flushToDisk {
storeChanges()
}
}
func removeAll() {
let tabs = self.tabs
for tab in tabs {
self.removeTab(tab, flushToDisk: false)
}
storeChanges()
}
func getIndex(tab: Browser) -> Int {
for i in 0..<count {
if tabs[i] === tab {
return i
}
}
assertionFailure("Tab not in tabs list")
return -1
}
private func storeChanges() {
// It is possible that not all tabs have loaded yet, so we filter out tabs with a nil URL.
let storedTabs: [RemoteTab] = optFilter(tabs.map(Browser.toTab))
self.profile.storeTabs(storedTabs)
// Also save (full) tab state to disk
preserveTabs()
}
func prefsDidChange() {
let allowPopups = !(self.profile.prefs.boolForKey("blockPopups") ?? true)
for tab in tabs {
tab.webView?.configuration.preferences.javaScriptCanOpenWindowsAutomatically = allowPopups
}
}
}
extension TabManager {
class SavedTab: NSObject, NSCoding {
let isSelected: Bool
let screenshot: UIImage?
var sessionData: SessionData?
init?(browser: Browser, isSelected: Bool) {
let currentItem = browser.webView?.backForwardList.currentItem
if browser.sessionData == nil {
let backList = browser.webView?.backForwardList.backList as? [WKBackForwardListItem] ?? []
let forwardList = browser.webView?.backForwardList.forwardList as? [WKBackForwardListItem] ?? []
let currentList = (currentItem != nil) ? [currentItem!] : []
var urlList = backList + currentList + forwardList
var updatedUrlList = [NSURL]()
for url in urlList {
updatedUrlList.append(url.URL)
}
var currentPage = -forwardList.count
self.sessionData = SessionData(currentPage: currentPage, urls: updatedUrlList)
} else {
self.sessionData = browser.sessionData
}
self.screenshot = browser.screenshot
self.isSelected = isSelected
super.init()
}
required init(coder: NSCoder) {
self.sessionData = coder.decodeObjectForKey("sessionData") as? SessionData
self.screenshot = coder.decodeObjectForKey("screenshot") as? UIImage
self.isSelected = coder.decodeBoolForKey("isSelected")
}
func encodeWithCoder(coder: NSCoder) {
coder.encodeObject(sessionData, forKey: "sessionData")
coder.encodeObject(screenshot, forKey: "screenshot")
coder.encodeBool(isSelected, forKey: "isSelected")
}
}
private func tabsStateArchivePath() -> String? {
if let documentsPath = NSSearchPathForDirectoriesInDomains(.DocumentDirectory, .UserDomainMask, true)[0] as? String {
return documentsPath.stringByAppendingPathComponent("tabsState.archive")
}
return nil
}
private func preserveTabsInternal() {
if let path = tabsStateArchivePath() {
var savedTabs = [SavedTab]()
for (tabIndex, tab) in enumerate(tabs) {
if let savedTab = SavedTab(browser: tab, isSelected: tabIndex == selectedIndex) {
savedTabs.append(savedTab)
}
}
let tabStateData = NSMutableData()
let archiver = NSKeyedArchiver(forWritingWithMutableData: tabStateData)
archiver.encodeObject(savedTabs, forKey: "tabs")
archiver.finishEncoding()
tabStateData.writeToFile(path, atomically: true)
}
}
func preserveTabs() {
// This is wrapped in an Objective-C @try/@catch handler because NSKeyedArchiver may throw exceptions which Swift cannot handle
Try(
try: { () -> Void in
self.preserveTabsInternal()
},
catch: { exception in
println("Failed to preserve tabs: \(exception)")
}
)
}
private func restoreTabsInternal() {
if let tabStateArchivePath = tabsStateArchivePath() {
if NSFileManager.defaultManager().fileExistsAtPath(tabStateArchivePath) {
if let data = NSData(contentsOfFile: tabStateArchivePath) {
let unarchiver = NSKeyedUnarchiver(forReadingWithData: data)
if let savedTabs = unarchiver.decodeObjectForKey("tabs") as? [SavedTab] {
var tabToSelect: Browser?
for (tabIndex, savedTab) in enumerate(savedTabs) {
let tab = self.addTab(flushToDisk: false, zombie: true, restoring: true)
tab.screenshot = savedTab.screenshot
if savedTab.isSelected {
tabToSelect = tab
}
tab.sessionData = savedTab.sessionData
}
if tabToSelect == nil {
tabToSelect = tabs.first
}
for delegate in delegates {
delegate.get()?.tabManagerDidRestoreTabs(self)
}
if let tab = tabToSelect {
selectTab(tab)
tab.createWebview()
}
}
}
}
}
}
func restoreTabs() {
// This is wrapped in an Objective-C @try/@catch handler because NSKeyedUnarchiver may throw exceptions which Swift cannot handle
Try(
try: { () -> Void in
self.restoreTabsInternal()
},
catch: { exception in
println("Failed to restore tabs: \(exception)")
}
)
}
}
extension TabManager : WKNavigationDelegate {
func webView(webView: WKWebView, didStartProvisionalNavigation navigation: WKNavigation!) {
UIApplication.sharedApplication().networkActivityIndicatorVisible = true
}
func webView(webView: WKWebView, didFinishNavigation navigation: WKNavigation!) {
hideNetworkActivitySpinner()
storeChanges()
}
func webView(webView: WKWebView, didFailNavigation navigation: WKNavigation!, withError error: NSError) {
hideNetworkActivitySpinner()
}
func hideNetworkActivitySpinner() {
for tab in tabs {
if let tabWebView = tab.webView {
// If we find one tab loading, we don't hide the spinner
if tabWebView.loading {
return
}
}
}
UIApplication.sharedApplication().networkActivityIndicatorVisible = false
}
}
// WKNavigationDelegates must implement NSObjectProtocol
class TabManagerNavDelegate : NSObject, WKNavigationDelegate {
private var delegates = WeakList<WKNavigationDelegate>()
func insert(delegate: WKNavigationDelegate) {
delegates.insert(delegate)
}
func webView(webView: WKWebView, didCommitNavigation navigation: WKNavigation!) {
for delegate in delegates {
delegate.webView?(webView, didCommitNavigation: navigation)
}
}
func webView(webView: WKWebView, didFailNavigation navigation: WKNavigation!, withError error: NSError) {
for delegate in delegates {
delegate.webView?(webView, didFailNavigation: navigation, withError: error)
}
}
func webView(webView: WKWebView, didFailProvisionalNavigation navigation: WKNavigation!,
withError error: NSError) {
for delegate in delegates {
delegate.webView?(webView, didFailProvisionalNavigation: navigation, withError: error)
}
}
func webView(webView: WKWebView, didFinishNavigation navigation: WKNavigation!) {
for delegate in delegates {
delegate.webView?(webView, didFinishNavigation: navigation)
}
}
func webView(webView: WKWebView, didReceiveAuthenticationChallenge challenge: NSURLAuthenticationChallenge,
completionHandler: (NSURLSessionAuthChallengeDisposition,
NSURLCredential!) -> Void) {
var disp: NSURLSessionAuthChallengeDisposition? = nil
for delegate in delegates {
delegate.webView?(webView, didReceiveAuthenticationChallenge: challenge) { (disposition, credential) in
// Whoever calls this method first wins. All other calls are ignored.
if disp != nil {
return
}
disp = disposition
completionHandler(disposition, credential)
}
}
}
func webView(webView: WKWebView, didReceiveServerRedirectForProvisionalNavigation navigation: WKNavigation!) {
for delegate in delegates {
delegate.webView?(webView, didReceiveServerRedirectForProvisionalNavigation: navigation)
}
}
func webView(webView: WKWebView, didStartProvisionalNavigation navigation: WKNavigation!) {
for delegate in delegates {
delegate.webView?(webView, didStartProvisionalNavigation: navigation)
}
}
func webView(webView: WKWebView, decidePolicyForNavigationAction navigationAction: WKNavigationAction,
decisionHandler: (WKNavigationActionPolicy) -> Void) {
var res = WKNavigationActionPolicy.Allow
for delegate in delegates {
delegate.webView?(webView, decidePolicyForNavigationAction: navigationAction, decisionHandler: { policy in
if policy == .Cancel {
res = policy
}
})
}
decisionHandler(res)
}
func webView(webView: WKWebView, decidePolicyForNavigationResponse navigationResponse: WKNavigationResponse,
decisionHandler: (WKNavigationResponsePolicy) -> Void) {
var res = WKNavigationResponsePolicy.Allow
for delegate in delegates {
delegate.webView?(webView, decidePolicyForNavigationResponse: navigationResponse, decisionHandler: { policy in
if policy == .Cancel {
res = policy
}
})
}
decisionHandler(res)
}
}
| mpl-2.0 | 103978b5c97e080682824b81e2353f46 | 33.633205 | 166 | 0.598997 | 5.668246 | false | false | false | false |
julienbodet/wikipedia-ios | TopReadWidget/WMFTodayTopReadWidgetViewController.swift | 1 | 16611 | import UIKit
import NotificationCenter
import WMF
class WMFTodayTopReadWidgetViewController: UIViewController, NCWidgetProviding {
// Model
var siteURL: URL!
var groupURL: URL?
var results: [WMFFeedTopReadArticlePreview] = []
var feedContentFetcher = WMFFeedContentFetcher()
var userStore: MWKDataStore!
var contentSource: WMFFeedContentSource!
let databaseDateFormatter = DateFormatter.wmf_englishUTCNonDelimitedYearMonthDay()
let headerDateFormatter = DateFormatter.wmf_shortMonthNameDayOfMonthNumber()
let daysToShowInSparkline = 5
@IBOutlet weak var footerLabelLeadingConstraint: NSLayoutConstraint!
@IBOutlet weak var headerLabelLeadingConstraint: NSLayoutConstraint!
@IBOutlet weak var activityIndicatorView: UIActivityIndicatorView!
#if DEBUG
let skipCache = false
#else
let skipCache = false
#endif
// Views & View State
@IBOutlet weak var headerView: UIView!
@IBOutlet weak var headerLabel: UILabel!
@IBOutlet weak var footerView: UIView!
@IBOutlet weak var footerLabel: UILabel!
@IBOutlet weak var footerViewBottomConstraint: NSLayoutConstraint!
@IBOutlet weak var footerViewHeightConstraint: NSLayoutConstraint!
@IBOutlet weak var headerViewHeightConstraint: NSLayoutConstraint!
@IBOutlet weak var headerViewTopConstraint: NSLayoutConstraint!
@IBOutlet weak var stackViewWidthConstraint: NSLayoutConstraint!
@IBOutlet var stackViewHeightConstraint: NSLayoutConstraint!
@IBOutlet weak var stackViewTopConstraint: NSLayoutConstraint!
@IBOutlet weak var footerSeparatorView: UIView!
@IBOutlet weak var headerSeparatorView: UIView!
@IBOutlet weak var stackView: UIStackView!
let cellReuseIdentifier = "articleList"
let maximumRowCount = 3
var maximumSize = CGSize.zero
var rowCount = 3
var footerVisible = true
var headerVisible = true
var isExpanded: Bool?
// Controllers
var articlePreviewViewControllers: [WMFArticlePreviewViewController] = []
var theme: Theme = .widget
override func viewDidLoad() {
super.viewDidLoad()
guard let appLanguage = MWKLanguageLinkController.sharedInstance().appLanguage else {
return
}
siteURL = appLanguage.siteURL()
userStore = SessionSingleton.sharedInstance().dataStore
contentSource = WMFFeedContentSource(siteURL: siteURL, userDataStore: userStore, notificationsController: nil)
let tapGR = UITapGestureRecognizer(target: self, action: #selector(self.handleTapGestureRecognizer(_:)))
view.addGestureRecognizer(tapGR)
extensionContext?.widgetLargestAvailableDisplayMode = .expanded
}
func layoutForSize(_ size: CGSize) {
let headerHeight = headerViewHeightConstraint.constant
headerViewTopConstraint.constant = headerVisible ? 0 : 0 - headerHeight
stackViewTopConstraint.constant = headerVisible ? headerHeight : 0
stackViewWidthConstraint.constant = size.width
var i = 0
for vc in articlePreviewViewControllers {
vc.view.alpha = i < rowCount ? 1 : 0
if i == 0 {
vc.separatorView.alpha = rowCount == 1 ? 0 : 1
}
i += 1
}
footerView.alpha = footerVisible ? 1 : 0
headerView.alpha = headerVisible ? 1 : 0
view.layoutIfNeeded()
}
override func viewWillTransition(to size: CGSize, with coordinator: UIViewControllerTransitionCoordinator) {
super.viewWillTransition(to: size, with: coordinator)
coordinator.animate(alongsideTransition: { (context) in
self.layoutForSize(size)
}) { (context) in
if (!context.isAnimated) {
self.layoutForSize(size)
}
}
}
func updateViewPropertiesForIsExpanded(_ isExpanded: Bool) {
self.isExpanded = isExpanded
headerVisible = isExpanded
footerVisible = headerVisible
rowCount = isExpanded ? maximumRowCount : 1
}
func widgetActiveDisplayModeDidChange(_ activeDisplayMode: NCWidgetDisplayMode, withMaximumSize maxSize: CGSize) {
debounceViewUpdate()
}
func debounceViewUpdate() {
NSObject.cancelPreviousPerformRequests(withTarget: self, selector: #selector(updateView), object: nil)
perform(#selector(updateView), with: nil, afterDelay: 0.1)
}
@objc func updateView() {
guard viewIfLoaded != nil else {
return
}
if let context = self.extensionContext {
var updatedIsExpanded: Bool?
updatedIsExpanded = context.widgetActiveDisplayMode == .expanded
maximumSize = context.widgetMaximumSize(for: context.widgetActiveDisplayMode)
if isExpanded != updatedIsExpanded {
isExpanded = updatedIsExpanded
updateViewPropertiesForIsExpanded(isExpanded ?? false)
layoutForSize(view.bounds.size)
}
}
let count = min(results.count, maximumRowCount)
guard count > 0 else {
return
}
var language: String? = nil
let siteURL = self.siteURL as NSURL
if let languageCode = siteURL.wmf_language {
language = (Locale.current as NSLocale).wmf_localizedLanguageNameForCode(languageCode)
}
var headerText = ""
if let language = language {
headerText = String.localizedStringWithFormat(WMFLocalizedString("top-read-header-with-language", value:"%1$@ Wikipedia", comment: "%1$@ Wikipedia - for example English Wikipedia\n{{Identical|Wikipedia}}"), language)
} else {
headerText = WMFLocalizedString("top-read-header-generic", value:"Wikipedia", comment: "Wikipedia\n{{Identical|Wikipedia}}")
}
headerLabel.textColor = theme.colors.primaryText
headerLabel.text = headerText.uppercased()
headerLabel.isAccessibilityElement = false
footerLabel.text = WMFLocalizedString("top-read-see-more", value:"See more top read", comment: "Text for footer button allowing the user to see more top read articles").uppercased()
footerLabel.textColor = theme.colors.primaryText
var dataValueMin = CGFloat.greatestFiniteMagnitude
var dataValueMax = CGFloat.leastNormalMagnitude
for result in results[0...(maximumRowCount - 1)] {
let articlePreview = self.userStore.fetchArticle(with: result.articleURL)
guard let dataValues = articlePreview?.pageViews else {
continue
}
for (_, dataValue) in dataValues {
guard let number = dataValue as? NSNumber else {
continue
}
let floatValue = CGFloat(number.doubleValue)
if (floatValue < dataValueMin) {
dataValueMin = floatValue
}
if (floatValue > dataValueMax) {
dataValueMax = floatValue
}
}
}
headerSeparatorView.backgroundColor = theme.colors.border
footerSeparatorView.backgroundColor = theme.colors.border
var i = 0
while i < count {
var vc: WMFArticlePreviewViewController
if (i < articlePreviewViewControllers.count) {
vc = articlePreviewViewControllers[i]
} else {
vc = WMFArticlePreviewViewController()
articlePreviewViewControllers.append(vc)
}
if vc.parent == nil {
addChildViewController(vc)
stackView.addArrangedSubview(vc.view)
vc.didMove(toParentViewController: self)
}
let result = results[i]
vc.titleTextColor = theme.colors.primaryText
vc.subtitleLabel.textColor = theme.colors.secondaryText
vc.rankLabel.textColor = theme.colors.secondaryText
vc.viewCountLabel.textColor = theme.colors.overlayText
vc.titleHTML = result.displayTitleHTML
if let wikidataDescription = result.wikidataDescription {
vc.subtitleLabel.text = wikidataDescription.wmf_stringByCapitalizingFirstCharacter(usingWikipediaLanguage: siteURL.wmf_language)
}else{
vc.subtitleLabel.text = result.snippet
}
vc.imageView.wmf_reset()
let rankString = NumberFormatter.localizedThousandsStringFromNumber(NSNumber(value: i + 1))
vc.rankLabel.text = rankString
vc.rankLabel.accessibilityLabel = String.localizedStringWithFormat(WMFLocalizedString("rank-accessibility-label", value:"Number %1$@", comment: "Accessibility label read aloud to sight impared users to indicate a ranking - Number 1, Number 2, etc. %1$@ is replaced with the ranking\n{{Identical|Number}}"), rankString)
if let articlePreview = self.userStore.fetchArticle(with: result.articleURL) {
vc.viewCountAndSparklineContainerView.backgroundColor = theme.colors.overlayBackground
if var viewCounts = articlePreview.pageViewsSortedByDate, viewCounts.count >= daysToShowInSparkline {
vc.sparklineView.minDataValue = dataValueMin
vc.sparklineView.maxDataValue = dataValueMax
let countToRemove = viewCounts.count - daysToShowInSparkline
if countToRemove > 0 {
viewCounts.removeFirst(countToRemove)
}
vc.sparklineView.dataValues = viewCounts
if let count = viewCounts.last {
vc.viewCountLabel.text = NumberFormatter.localizedThousandsStringFromNumber(count)
if let numberString = NumberFormatter.threeSignificantDigitWholeNumberFormatter.string(from: count) {
let format = WMFLocalizedString("readers-accessibility-label", value:"%1$@ readers", comment: "Accessibility label read aloud to sight impared users to indicate number of readers for a given article - %1$@ is replaced with the number of readers\n{{Identical|Reader}}")
vc.viewCountLabel.accessibilityLabel = String.localizedStringWithFormat(format,numberString)
}
} else {
vc.viewCountLabel.accessibilityLabel = nil
vc.viewCountLabel.text = nil
}
vc.viewCountAndSparklineContainerView.isHidden = false
} else {
vc.viewCountAndSparklineContainerView.isHidden = true
}
} else {
vc.viewCountAndSparklineContainerView.isHidden = true
}
if let imageURL = result.thumbnailURL {
vc.imageView.wmf_setImage(with: imageURL, detectFaces: true, onGPU: true, failure: { (error) in
vc.collapseImageAndWidenLabels = true
}) {
vc.collapseImageAndWidenLabels = false
}
} else {
vc.collapseImageAndWidenLabels = true
}
if i == (count - 1) {
vc.separatorView.isHidden = true
} else {
vc.separatorView.isHidden = false
}
vc.separatorView.backgroundColor = theme.colors.border
i += 1
}
stackViewHeightConstraint.isActive = false
stackViewWidthConstraint.constant = maximumSize.width
var sizeToFit = UILayoutFittingCompressedSize
sizeToFit.width = maximumSize.width
var size = stackView.systemLayoutSizeFitting(sizeToFit, withHorizontalFittingPriority: UILayoutPriority.required, verticalFittingPriority: UILayoutPriority.defaultLow)
size.width = maximumSize.width
stackViewHeightConstraint.isActive = true
stackViewHeightConstraint.constant = size.height
view.layoutIfNeeded()
let headerHeight = headerViewHeightConstraint.constant
let footerHeight = footerViewHeightConstraint.constant
if headerVisible {
size.height += headerHeight
}
if footerVisible {
size.height += footerHeight
}
preferredContentSize = rowCount == 1 ? articlePreviewViewControllers[0].view.frame.size : size
activityIndicatorHidden = true
}
var activityIndicatorHidden: Bool = false {
didSet {
activityIndicatorView.isHidden = activityIndicatorHidden
if activityIndicatorHidden {
activityIndicatorView.stopAnimating()
} else {
activityIndicatorView.startAnimating()
}
headerView.isHidden = !activityIndicatorHidden
footerView.isHidden = !activityIndicatorHidden
stackView.isHidden = !activityIndicatorHidden
}
}
func widgetPerformUpdate(completionHandler: @escaping (NCUpdateResult) -> Void) {
fetch(siteURL: siteURL, date:Date(), attempt: 1, completionHandler: completionHandler)
}
func updateUIWithTopReadFromContentStoreForSiteURL(siteURL: URL, date: Date) -> NCUpdateResult {
if let topRead = self.userStore.viewContext.group(of: .topRead, for: date, siteURL: siteURL) {
if let content = topRead.contentPreview as? [WMFFeedTopReadArticlePreview] {
if let previousGroupURL = self.groupURL,
let topReadURL = topRead.url,
self.results.count > 0,
previousGroupURL == topReadURL {
return .noData
}
self.groupURL = topRead.url
self.results = content
self.updateView()
return .newData
}
}
return .failed
}
func fetch(siteURL: URL, date: Date, attempt: Int, completionHandler: @escaping ((NCUpdateResult) -> Void)) {
let result = updateUIWithTopReadFromContentStoreForSiteURL(siteURL: siteURL, date: date)
guard result == .failed else {
completionHandler(result)
return
}
guard attempt < 4 else {
completionHandler(.noData)
return
}
contentSource.loadContent(for: date, in: userStore.viewContext, force: false) {
DispatchQueue.main.async(execute: {
let result = self.updateUIWithTopReadFromContentStoreForSiteURL(siteURL: siteURL, date: date)
guard result != .failed else {
if (attempt == 1) {
let todayUTC = (date as NSDate).wmf_midnightLocalDateForEquivalentUTC as Date
self.fetch(siteURL: siteURL, date: todayUTC, attempt: attempt + 1, completionHandler: completionHandler)
} else {
guard let previousDate = NSCalendar.wmf_gregorian().date(byAdding: .day, value: -1, to: date, options: .matchStrictly) else {
completionHandler(.noData)
return
}
self.fetch(siteURL: siteURL, date: previousDate, attempt: attempt + 1, completionHandler: completionHandler)
}
return
}
completionHandler(result)
})
}
}
func showAllTopReadInApp() {
guard let URL = groupURL else {
return
}
self.extensionContext?.open(URL)
}
@objc func handleTapGestureRecognizer(_ gestureRecognizer: UITapGestureRecognizer) {
guard let index = self.articlePreviewViewControllers.index(where: { (vc) -> Bool in
let convertedRect = self.view.convert(vc.view.frame, from: vc.view.superview)
return convertedRect.contains(gestureRecognizer.location(in: self.view))
}), index < results.count else {
showAllTopReadInApp()
return
}
let result = results[index]
self.extensionContext?.open(result.articleURL)
}
}
| mit | 37136e81e55fd8cc950a1b871593ed5a | 40.94697 | 330 | 0.617242 | 5.644241 | false | false | false | false |
dshahidehpour/IGListKit | Examples/Examples-iOS/IGListKitExamples/Views/RemoveCell.swift | 4 | 1876 | /**
Copyright (c) 2016-present, Facebook, Inc. All rights reserved.
The examples provided by Facebook are for non-commercial testing and evaluation
purposes only. Facebook reserves all rights not expressly granted.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL
FACEBOOK BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN
ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*/
import UIKit
protocol RemoveCellDelegate: class {
func removeCellDidTapButton(_ cell: RemoveCell)
}
class RemoveCell: UICollectionViewCell {
weak var delegate: RemoveCellDelegate?
lazy var label: UILabel = {
let label = UILabel()
label.backgroundColor = .clear
self.contentView.addSubview(label)
return label
}()
fileprivate lazy var button: UIButton = {
let button = UIButton(type: .custom)
button.setTitle("Remove", for: UIControlState())
button.setTitleColor(.blue, for: UIControlState())
button.backgroundColor = .clear
button.addTarget(self, action: #selector(RemoveCell.onButton(_:)), for: .touchUpInside)
self.contentView.addSubview(button)
return button
}()
override func layoutSubviews() {
super.layoutSubviews()
contentView.backgroundColor = .white
let bounds = contentView.bounds
let divide = bounds.divided(atDistance: 100, from: .maxXEdge)
label.frame = divide.slice.insetBy(dx: 15, dy: 0)
button.frame = divide.remainder
}
func onButton(_ button: UIButton) {
delegate?.removeCellDidTapButton(self)
}
}
| bsd-3-clause | 5ec4a3cc79e2a9e50ebe1b507277c51b | 33.109091 | 95 | 0.702026 | 4.773537 | false | false | false | false |
parkera/swift-corelibs-foundation | Foundation/Scanner.swift | 1 | 27846 | // This source file is part of the Swift.org open source project
//
// Copyright (c) 2014 - 2016 Apple Inc. and the Swift project authors
// Licensed under Apache License v2.0 with Runtime Library Exception
//
// See http://swift.org/LICENSE.txt for license information
// See http://swift.org/CONTRIBUTORS.txt for the list of Swift project authors
//
import CoreFoundation
open class Scanner: NSObject, NSCopying {
internal var _scanString: String
internal var _skipSet: CharacterSet?
internal var _invertedSkipSet: CharacterSet?
internal var _scanLocation: Int
open override func copy() -> Any {
return copy(with: nil)
}
open func copy(with zone: NSZone? = nil) -> Any {
return Scanner(string: string)
}
open var string: String {
return _scanString
}
open var scanLocation: Int {
get {
return _scanLocation
}
set {
if newValue > string.length {
fatalError("Index \(newValue) beyond bounds; string length \(string.length)")
}
_scanLocation = newValue
}
}
/*@NSCopying*/ open var charactersToBeSkipped: CharacterSet? {
get {
return _skipSet
}
set {
_skipSet = newValue
_invertedSkipSet = nil
}
}
internal var invertedSkipSet: CharacterSet? {
if let inverted = _invertedSkipSet {
return inverted
} else {
if let set = charactersToBeSkipped {
_invertedSkipSet = set.inverted
return _invertedSkipSet
}
return nil
}
}
open var caseSensitive: Bool = false
open var locale: Any?
internal static let defaultSkipSet = CharacterSet.whitespacesAndNewlines
public init(string: String) {
_scanString = string
_skipSet = Scanner.defaultSkipSet
_scanLocation = 0
}
// On overflow, the below methods will return success and clamp
@discardableResult
open func scanInt32(_ result: UnsafeMutablePointer<Int32>) -> Bool {
return _scanString.scan(_skipSet, locationToScanFrom: &_scanLocation) { (value: Int32) -> Void in
result.pointee = value
}
}
@discardableResult
open func scanInt(_ result: UnsafeMutablePointer<Int>) -> Bool {
return _scanString.scan(_skipSet, locationToScanFrom: &_scanLocation) { (value: Int) -> Void in
result.pointee = value
}
}
@discardableResult
open func scanInt64(_ result: UnsafeMutablePointer<Int64>) -> Bool {
return _scanString.scan(_skipSet, locationToScanFrom: &_scanLocation) { (value: Int64) -> Void in
result.pointee = value
}
}
@discardableResult
open func scanUnsignedLongLong(_ result: UnsafeMutablePointer<UInt64>) -> Bool {
return _scanString.scan(_skipSet, locationToScanFrom: &_scanLocation) { (value: UInt64) -> Void in
result.pointee = value
}
}
@discardableResult
open func scanFloat(_ result: UnsafeMutablePointer<Float>) -> Bool {
return _scanString.scan(_skipSet, locale: locale as? Locale, locationToScanFrom: &_scanLocation) { (value: Float) -> Void in
result.pointee = value
}
}
@discardableResult
open func scanDouble(_ result: UnsafeMutablePointer<Double>) -> Bool {
return _scanString.scan(_skipSet, locale: locale as? Locale, locationToScanFrom: &_scanLocation) { (value: Double) -> Void in
result.pointee = value
}
}
@discardableResult
open func scanHexInt32(_ result: UnsafeMutablePointer<UInt32>) -> Bool {
return _scanString.scanHex(_skipSet, locationToScanFrom: &_scanLocation) { (value: UInt32) -> Void in
result.pointee = value
}
}
@discardableResult
open func scanHexInt64(_ result: UnsafeMutablePointer<UInt64>) -> Bool {
return _scanString.scanHex(_skipSet, locationToScanFrom: &_scanLocation) { (value: UInt64) -> Void in
result.pointee = value
}
}
@discardableResult
open func scanHexFloat(_ result: UnsafeMutablePointer<Float>) -> Bool {
return _scanString.scanHex(_skipSet, locale: locale as? Locale, locationToScanFrom: &_scanLocation) { (value: Float) -> Void in
result.pointee = value
}
}
@discardableResult
open func scanHexDouble(_ result: UnsafeMutablePointer<Double>) -> Bool {
return _scanString.scanHex(_skipSet, locale: locale as? Locale, locationToScanFrom: &_scanLocation) { (value: Double) -> Void in
result.pointee = value
}
}
open var isAtEnd: Bool {
var stringLoc = scanLocation
let stringLen = string.length
if let invSet = invertedSkipSet {
let range = string._nsObject.rangeOfCharacter(from: invSet, options: [], range: NSRange(location: stringLoc, length: stringLen - stringLoc))
stringLoc = range.length > 0 ? range.location : stringLen
}
return stringLoc == stringLen
}
open class func localizedScanner(with string: String) -> Any {
let scanner = Scanner(string: string)
scanner.locale = Locale.current
return scanner
}
}
internal struct _NSStringBuffer {
var bufferLen: Int
var bufferLoc: Int
var string: NSString
var stringLen: Int
var _stringLoc: Int
var buffer = Array<unichar>(repeating: 0, count: 32)
var curChar: unichar?
static let EndCharacter = unichar(0xffff)
init(string: String, start: Int, end: Int) {
self.string = string._bridgeToObjectiveC()
_stringLoc = start
stringLen = end
if _stringLoc < stringLen {
bufferLen = min(32, stringLen - _stringLoc)
let range = NSRange(location: _stringLoc, length: bufferLen)
bufferLoc = 1
buffer.withUnsafeMutableBufferPointer({ (ptr: inout UnsafeMutableBufferPointer<unichar>) -> Void in
self.string.getCharacters(ptr.baseAddress!, range: range)
})
curChar = buffer[0]
} else {
bufferLen = 0
bufferLoc = 1
curChar = _NSStringBuffer.EndCharacter
}
}
init(string: NSString, start: Int, end: Int) {
self.string = string
_stringLoc = start
stringLen = end
if _stringLoc < stringLen {
bufferLen = min(32, stringLen - _stringLoc)
let range = NSRange(location: _stringLoc, length: bufferLen)
bufferLoc = 1
buffer.withUnsafeMutableBufferPointer({ (ptr: inout UnsafeMutableBufferPointer<unichar>) -> Void in
self.string.getCharacters(ptr.baseAddress!, range: range)
})
curChar = buffer[0]
} else {
bufferLen = 0
bufferLoc = 1
curChar = _NSStringBuffer.EndCharacter
}
}
var currentCharacter: unichar {
return curChar!
}
var isAtEnd: Bool {
return curChar == _NSStringBuffer.EndCharacter
}
mutating func fill() {
bufferLen = min(32, stringLen - _stringLoc)
let range = NSRange(location: _stringLoc, length: bufferLen)
buffer.withUnsafeMutableBufferPointer({ (ptr: inout UnsafeMutableBufferPointer<unichar>) -> Void in
string.getCharacters(ptr.baseAddress!, range: range)
})
bufferLoc = 1
curChar = buffer[0]
}
mutating func advance() {
if bufferLoc < bufferLen { /*buffer is OK*/
curChar = buffer[bufferLoc]
bufferLoc += 1
} else if (_stringLoc + bufferLen < stringLen) { /* Buffer is empty but can be filled */
_stringLoc += bufferLen
fill()
} else { /* Buffer is empty and we're at the end */
bufferLoc = bufferLen + 1
curChar = _NSStringBuffer.EndCharacter
}
}
mutating func rewind() {
if bufferLoc > 1 { /* Buffer is OK */
bufferLoc -= 1
curChar = buffer[bufferLoc - 1]
} else if _stringLoc > 0 { /* Buffer is empty but can be filled */
bufferLoc = min(32, _stringLoc)
bufferLen = bufferLoc
_stringLoc -= bufferLen
let range = NSRange(location: _stringLoc, length: bufferLen)
buffer.withUnsafeMutableBufferPointer({ (ptr: inout UnsafeMutableBufferPointer<unichar>) -> Void in
string.getCharacters(ptr.baseAddress!, range: range)
})
curChar = buffer[bufferLoc - 1]
} else {
bufferLoc = 0
curChar = _NSStringBuffer.EndCharacter
}
}
mutating func skip(_ skipSet: CharacterSet?) {
if let set = skipSet {
while set.contains(UnicodeScalar(currentCharacter)!) && !isAtEnd {
advance()
}
}
}
var location: Int {
get {
return _stringLoc + bufferLoc - 1
}
mutating set {
if newValue < _stringLoc || newValue >= _stringLoc + bufferLen {
if newValue < 16 { /* Get the first NSStringBufferSize chars */
_stringLoc = 0
} else if newValue > stringLen - 16 { /* Get the last NSStringBufferSize chars */
_stringLoc = stringLen < 32 ? 0 : stringLen - 32
} else {
_stringLoc = newValue - 16 /* Center around loc */
}
fill()
}
bufferLoc = newValue - _stringLoc
curChar = buffer[bufferLoc]
bufferLoc += 1
}
}
}
private func isADigit(_ ch: unichar) -> Bool {
struct Local {
static let set = CharacterSet.decimalDigits
}
return Local.set.contains(UnicodeScalar(ch)!)
}
private func numericValue(_ ch: unichar) -> Int {
if (ch >= unichar(unicodeScalarLiteral: "0") && ch <= unichar(unicodeScalarLiteral: "9")) {
return Int(ch) - Int(unichar(unicodeScalarLiteral: "0"))
} else {
return __CFCharDigitValue(UniChar(ch))
}
}
private func numericOrHexValue(_ ch: unichar) -> Int {
if (ch >= unichar(unicodeScalarLiteral: "0") && ch <= unichar(unicodeScalarLiteral: "9")) {
return Int(ch) - Int(unichar(unicodeScalarLiteral: "0"))
} else if (ch >= unichar(unicodeScalarLiteral: "A") && ch <= unichar(unicodeScalarLiteral: "F")) {
return Int(ch) + 10 - Int(unichar(unicodeScalarLiteral: "A"))
} else if (ch >= unichar(unicodeScalarLiteral: "a") && ch <= unichar(unicodeScalarLiteral: "f")) {
return Int(ch) + 10 - Int(unichar(unicodeScalarLiteral: "a"))
} else {
return -1
}
}
private func decimalSep(_ locale: Locale?) -> String {
if let loc = locale {
if let sep = loc._bridgeToObjectiveC().object(forKey: .decimalSeparator) as? NSString {
return sep._swiftObject
}
return "."
} else {
return decimalSep(Locale.current)
}
}
extension String {
internal func scan<T: FixedWidthInteger>(_ skipSet: CharacterSet?, locationToScanFrom: inout Int, to: (T) -> Void) -> Bool {
var buf = _NSStringBuffer(string: self, start: locationToScanFrom, end: length)
buf.skip(skipSet)
var neg = false
var localResult: T = 0
if buf.currentCharacter == unichar(unicodeScalarLiteral: "-") || buf.currentCharacter == unichar(unicodeScalarLiteral: "+") {
neg = buf.currentCharacter == unichar(unicodeScalarLiteral: "-")
buf.advance()
buf.skip(skipSet)
}
if (!isADigit(buf.currentCharacter)) {
return false
}
repeat {
let numeral = numericValue(buf.currentCharacter)
if numeral == -1 {
break
}
if (localResult >= T.max / 10) && ((localResult > T.max / 10) || T(numeral - (neg ? 1 : 0)) >= T.max - localResult * 10) {
// apply the clamps and advance past the ending of the buffer where there are still digits
localResult = neg ? T.min : T.max
neg = false
repeat {
buf.advance()
} while (isADigit(buf.currentCharacter))
break
} else {
// normal case for scanning
localResult = localResult * 10 + T(numeral)
}
buf.advance()
} while (isADigit(buf.currentCharacter))
to(neg ? -1 * localResult : localResult)
locationToScanFrom = buf.location
return true
}
internal func scanHex<T: FixedWidthInteger>(_ skipSet: CharacterSet?, locationToScanFrom: inout Int, to: (T) -> Void) -> Bool {
var buf = _NSStringBuffer(string: self, start: locationToScanFrom, end: length)
buf.skip(skipSet)
var localResult: T = 0
var curDigit: Int
if buf.currentCharacter == unichar(unicodeScalarLiteral: "0") {
buf.advance()
let locRewindTo = buf.location
curDigit = numericOrHexValue(buf.currentCharacter)
if curDigit == -1 {
if buf.currentCharacter == unichar(unicodeScalarLiteral: "x") || buf.currentCharacter == unichar(unicodeScalarLiteral: "X") {
buf.advance()
curDigit = numericOrHexValue(buf.currentCharacter)
}
}
if curDigit == -1 {
locationToScanFrom = locRewindTo
to(T(0))
return true
}
} else {
curDigit = numericOrHexValue(buf.currentCharacter)
if curDigit == -1 {
return false
}
}
repeat {
if localResult > T.max >> T(4) {
localResult = T.max
} else {
localResult = (localResult << T(4)) + T(curDigit)
}
buf.advance()
curDigit = numericOrHexValue(buf.currentCharacter)
} while (curDigit != -1)
to(localResult)
locationToScanFrom = buf.location
return true
}
internal func scan<T: BinaryFloatingPoint>(_ skipSet: CharacterSet?, locale: Locale?, locationToScanFrom: inout Int, to: (T) -> Void) -> Bool {
let ds_chars = decimalSep(locale).utf16
let ds = ds_chars[ds_chars.startIndex]
var buf = _NSStringBuffer(string: self, start: locationToScanFrom, end: length)
buf.skip(skipSet)
var neg = false
var localResult: T = T(0)
if buf.currentCharacter == unichar(unicodeScalarLiteral: "-") || buf.currentCharacter == unichar(unicodeScalarLiteral: "+") {
neg = buf.currentCharacter == unichar(unicodeScalarLiteral: "-")
buf.advance()
buf.skip(skipSet)
}
if (buf.currentCharacter != ds && !isADigit(buf.currentCharacter)) {
return false
}
repeat {
let numeral = numericValue(buf.currentCharacter)
if numeral == -1 {
break
}
// if (localResult >= T.greatestFiniteMagnitude / T(10)) && ((localResult > T.greatestFiniteMagnitude / T(10)) || T(numericValue(buf.currentCharacter) - (neg ? 1 : 0)) >= T.greatestFiniteMagnitude - localResult * T(10)) is evidently too complex; so break it down to more "edible chunks"
let limit1 = localResult >= T.greatestFiniteMagnitude / T(10)
let limit2 = localResult > T.greatestFiniteMagnitude / T(10)
let limit3 = T(numeral - (neg ? 1 : 0)) >= T.greatestFiniteMagnitude - localResult * T(10)
if (limit1) && (limit2 || limit3) {
// apply the clamps and advance past the ending of the buffer where there are still digits
localResult = neg ? -T.infinity : T.infinity
neg = false
repeat {
buf.advance()
} while (isADigit(buf.currentCharacter))
break
} else {
localResult = localResult * T(10) + T(numeral)
}
buf.advance()
} while (isADigit(buf.currentCharacter))
if buf.currentCharacter == ds {
var factor = T(0.1)
buf.advance()
repeat {
let numeral = numericValue(buf.currentCharacter)
if numeral == -1 {
break
}
localResult = localResult + T(numeral) * factor
factor = factor * T(0.1)
buf.advance()
} while (isADigit(buf.currentCharacter))
}
if buf.currentCharacter == unichar(unicodeScalarLiteral: "e") || buf.currentCharacter == unichar(unicodeScalarLiteral: "E") {
var exponent = Double(0)
var negExponent = false
buf.advance()
if buf.currentCharacter == unichar(unicodeScalarLiteral: "-") || buf.currentCharacter == unichar(unicodeScalarLiteral: "+") {
negExponent = buf.currentCharacter == unichar(unicodeScalarLiteral: "-")
buf.advance()
}
repeat {
let numeral = numericValue(buf.currentCharacter)
buf.advance()
if numeral == -1 {
break
}
exponent *= 10
exponent += Double(numeral)
} while (isADigit(buf.currentCharacter))
if exponent > 0 {
let multiplier = pow(10, exponent)
if negExponent {
localResult /= T(multiplier)
} else {
localResult *= T(multiplier)
}
}
}
to(neg ? T(-1) * localResult : localResult)
locationToScanFrom = buf.location
return true
}
internal func scanHex<T: BinaryFloatingPoint>(_ skipSet: CharacterSet?, locale: Locale?, locationToScanFrom: inout Int, to: (T) -> Void) -> Bool {
NSUnimplemented()
}
}
// This extension used to house the experimental API for Scanner. This is all deprecated in favor of API with newer semantics. Some of the experimental API have been promoted to full API with slightly different semantics; see ScannerAPI.swift.
extension Scanner {
// These methods are in a special bit of mess:
// - They used to exist here; but
// - They have all been replaced by methods called scan<Type>(representation:); but
// - The representation parameter has a default value, so scan<Type>() is still valid and has the same semantics as the below.
// This means that the new methods _aren't_ fully source compatible — most source will correctly pick up the new .scan<Type>(representation:) with the default, but things like let method = scanner.scanInt32 may or may not work any longer.
// Make sure that the signatures exist here so that in case the compiler would pick them up, we can direct people to the new ones. This should be rare.
// scanDecimal() is not among these methods and has not changed at all, though it has been promoted to non-experimental API.
@available(swift, obsoleted: 5.0, renamed: "scanInt(representation:)")
public func scanInt() -> Int? { return scanInt(representation: .decimal) }
@available(swift, obsoleted: 5.0, renamed: "scanInt32(representation:)")
public func scanInt32() -> Int32? { return scanInt32(representation: .decimal) }
@available(swift, obsoleted: 5.0, renamed: "scanInt64(representation:)")
public func scanInt64() -> Int64? { return scanInt64(representation: .decimal) }
@available(swift, obsoleted: 5.0, renamed: "scanUInt64(representation:)")
public func scanUInt64() -> UInt64? { return scanUInt64(representation: .decimal) }
@available(swift, obsoleted: 5.0, renamed: "scanFloat(representation:)")
public func scanFloat() -> Float? { return scanFloat(representation: .decimal) }
@available(swift, obsoleted: 5.0, renamed: "scanDouble(representation:)")
public func scanDouble() -> Double? { return scanDouble(representation: .decimal) }
// These existed but are now deprecated in favor of the new methods:
@available(swift, deprecated: 5.0, renamed: "scanUInt64(representation:)")
public func scanHexInt32() -> UInt32? {
guard let value = scanUInt64(representation: .hexadecimal) else { return nil }
return UInt32(min(value, UInt64(UInt32.max)))
}
@available(swift, deprecated: 5.0, renamed: "scanUInt64(representation:)")
public func scanHexInt64() -> UInt64? { return scanUInt64(representation: .hexadecimal) }
@available(swift, deprecated: 5.0, renamed: "scanFloat(representation:)")
public func scanHexFloat() -> Float? { return scanFloat(representation: .hexadecimal) }
@available(swift, deprecated: 5.0, renamed: "scanDouble(representation:)")
public func scanHexDouble() -> Double? { return scanDouble(representation: .hexadecimal) }
@available(swift, deprecated: 5.0, renamed: "scanString(_:)")
@discardableResult
public func scanString(_ string:String, into ptr: UnsafeMutablePointer<String?>?) -> Bool {
if let str = _scanStringSplittingGraphemes(string) {
ptr?.pointee = str
return true
}
return false
}
// These methods avoid calling the private API for _invertedSkipSet and manually re-construct them so that it is only usage of public API usage
// Future implementations on Darwin of these methods will likely be more optimized to take advantage of the cached values.
private func _scanStringSplittingGraphemes(_ searchString: String) -> String? {
let str = self.string._bridgeToObjectiveC()
var stringLoc = scanLocation
let stringLen = str.length
let options: NSString.CompareOptions = [caseSensitive ? [] : .caseInsensitive, .anchored]
if let invSkipSet = charactersToBeSkipped?.inverted {
let range = str.rangeOfCharacter(from: invSkipSet, options: [], range: NSRange(location: stringLoc, length: stringLen - stringLoc))
stringLoc = range.length > 0 ? range.location : stringLen
}
let range = str.range(of: searchString, options: options, range: NSRange(location: stringLoc, length: stringLen - stringLoc))
if range.length > 0 {
/* ??? Is the range below simply range? 99.9% of the time, and perhaps even 100% of the time... Hmm... */
let res = str.substring(with: NSRange(location: stringLoc, length: range.location + range.length - stringLoc))
scanLocation = range.location + range.length
return res
}
return nil
}
@available(swift, deprecated: 5.0, renamed: "scanCharacters(from:)")
public func scanCharactersFromSet(_ set: CharacterSet) -> String? {
return _scanCharactersSplittingGraphemes(from: set)
}
@available(swift, deprecated: 5.0, renamed: "scanCharacters(from:)")
public func scanCharacters(from set: CharacterSet, into ptr: UnsafeMutablePointer<String?>?) -> Bool {
if let str = _scanCharactersSplittingGraphemes(from: set) {
ptr?.pointee = str
return true
}
return false
}
private func _scanCharactersSplittingGraphemes(from set: CharacterSet) -> String? {
let str = self.string._bridgeToObjectiveC()
var stringLoc = scanLocation
let stringLen = str.length
let options: NSString.CompareOptions = caseSensitive ? [] : .caseInsensitive
if let invSkipSet = charactersToBeSkipped?.inverted {
let range = str.rangeOfCharacter(from: invSkipSet, options: [], range: NSRange(location: stringLoc, length: stringLen - stringLoc))
stringLoc = range.length > 0 ? range.location : stringLen
}
var range = str.rangeOfCharacter(from: set.inverted, options: options, range: NSRange(location: stringLoc, length: stringLen - stringLoc))
if range.length == 0 {
range.location = stringLen
}
if stringLoc != range.location {
let res = str.substring(with: NSRange(location: stringLoc, length: range.location - stringLoc))
scanLocation = range.location
return res
}
return nil
}
@available(swift, deprecated: 5.0, renamed: "scanUpToString(_:)")
@discardableResult
public func scanUpTo(_ string:String, into ptr: UnsafeMutablePointer<String?>?) -> Bool {
if let str = _scanUpToStringSplittingGraphemes(string) {
ptr?.pointee = str
return true
}
return false
}
public func _scanUpToStringSplittingGraphemes(_ string: String) -> String? {
let str = self.string._bridgeToObjectiveC()
var stringLoc = scanLocation
let stringLen = str.length
let options: NSString.CompareOptions = caseSensitive ? [] : .caseInsensitive
if let invSkipSet = charactersToBeSkipped?.inverted {
let range = str.rangeOfCharacter(from: invSkipSet, options: [], range: NSRange(location: stringLoc, length: stringLen - stringLoc))
stringLoc = range.length > 0 ? range.location : stringLen
}
var range = str.range(of: string, options: options, range: NSRange(location: stringLoc, length: stringLen - stringLoc))
if range.length == 0 {
range.location = stringLen
}
if stringLoc != range.location {
let res = str.substring(with: NSRange(location: stringLoc, length: range.location - stringLoc))
scanLocation = range.location
return res
}
return nil
}
@available(swift, deprecated: 5.0, renamed: "scanUpToCharacters(from:)")
@discardableResult
public func scanUpToCharacters(from set: CharacterSet, into ptr: UnsafeMutablePointer<String?>?) -> Bool {
if let result = _scanSplittingGraphemesUpToCharacters(from: set) {
ptr?.pointee = result
return true
}
return false
}
@available(swift, deprecated: 5.0, renamed: "scanUpToCharacters(from:)")
public func scanUpToCharactersFromSet(_ set: CharacterSet) -> String? {
return _scanSplittingGraphemesUpToCharacters(from: set)
}
private func _scanSplittingGraphemesUpToCharacters(from set: CharacterSet) -> String? {
let str = self.string._bridgeToObjectiveC()
var stringLoc = scanLocation
let stringLen = str.length
let options: NSString.CompareOptions = caseSensitive ? [] : .caseInsensitive
if let invSkipSet = charactersToBeSkipped?.inverted {
let range = str.rangeOfCharacter(from: invSkipSet, options: [], range: NSRange(location: stringLoc, length: stringLen - stringLoc))
stringLoc = range.length > 0 ? range.location : stringLen
}
var range = str.rangeOfCharacter(from: set, options: options, range: NSRange(location: stringLoc, length: stringLen - stringLoc))
if range.length == 0 {
range.location = stringLen
}
if stringLoc != range.location {
let res = str.substring(with: NSRange(location: stringLoc, length: range.location - stringLoc))
scanLocation = range.location
return res
}
return nil
}
}
| apache-2.0 | f559f6c56ae681d2ac373f78db83103c | 39.648175 | 299 | 0.597759 | 5.003414 | false | false | false | false |
paruckerr/weather-app-swift | Network/ViewController.swift | 1 | 2387 | //
// ViewController.swift
// Network
//
// Created by Eduardo Parucker on 27/10/14.
// Copyright (c) 2014 Eduardo Parucker. All rights reserved.
//
import UIKit
class ViewController: UIViewController {
private let apiKey = "aabf85d2d2cf25a6ab284191078c01d4"
@IBOutlet weak var iconView: UIImageView!
@IBOutlet weak var currentTimeLabel: UILabel!
@IBOutlet weak var temperatureLabel: UILabel!
@IBOutlet weak var humidityLabel: UILabel!
@IBOutlet weak var precipitationLabel: UILabel!
@IBOutlet weak var summaryLabel: UILabel!
override func viewDidLoad() {
super.viewDidLoad()
let baseUrl = NSURL(string: "https://api.forecast.io/forecast/\(apiKey)/")
let forecastURL = NSURL(string: "37.8267,-122.423", relativeToURL: baseUrl)
println(forecastURL)
let sharedSession = NSURLSession.sharedSession()
let downloadTask: NSURLSessionDownloadTask = sharedSession.downloadTaskWithURL(
forecastURL!,
completionHandler: {( location: NSURL!, response: NSURLResponse!, error: NSError!) -> Void in
if (error == nil) {
let dataObject = NSData(contentsOfURL: location)
let weatherDictionary: NSDictionary = NSJSONSerialization.JSONObjectWithData(dataObject!, options: nil, error: nil) as NSDictionary
let currentWeather = Current(weatherDictionary: weatherDictionary)
dispatch_async(dispatch_get_main_queue(), { () -> Void in
self.temperatureLabel.text = "\(currentWeather.temperature)"
self.iconView.image = currentWeather.icon!
self.currentTimeLabel.text = "At \(currentWeather.currentTime!) it is"
self.humidityLabel.text = "\(currentWeather.humidity)"
self.precipitationLabel.text = "\(currentWeather.precipProbability)"
self.summaryLabel.text = "\(currentWeather.summary)"
})
}
})
downloadTask.resume()
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
}
| mit | 89fe50232b3ec9787c43bc2ce1688641 | 38.131148 | 151 | 0.600754 | 5.5 | false | false | false | false |
zhuyunfeng1224/XiheMtxx | XiheMtxx/VC/EditImage/GrayView.swift | 1 | 736 | //
// GrayView.swift
// EasyCard
//
// Created by echo on 2017/2/21.
// Copyright © 2017年 羲和. All rights reserved.
//
import UIKit
class GrayView: UIView {
var clearFrame: CGRect = CGRect.zero {
didSet {
self.clearLayer.frame = self.bounds
self.clearLayer.clearFrame = self.clearFrame
self.clearLayer.setNeedsDisplay()
}
}
var clearLayer = CenterClearLayer()
override init(frame: CGRect) {
super.init(frame: frame)
self.clearLayer.frame = self.bounds
self.layer.addSublayer(self.clearLayer)
}
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
}
| mit | 6777830c2fdd769f4ddb69b4ff71896a | 21.78125 | 59 | 0.607682 | 3.919355 | false | false | false | false |
statusbits/HomeKit-Demo | BetterHomeKit/TriggerCreateViewController.swift | 1 | 5465 | //
// TriggerViewController.swift
// BetterHomeKit
//
// Created by Khaos Tian on 8/2/14.
// Copyright (c) 2014 Oltica. All rights reserved.
//
import UIKit
import HomeKit
class TriggerCreateViewController: UIViewController, UITextFieldDelegate {
weak var pendingTrigger:HMTimerTrigger?
@IBOutlet weak var datePicker: UIDatePicker!
@IBOutlet weak var nameField: UITextField!
@IBOutlet weak var repeatSwitch: UISwitch!
@IBOutlet weak var repeatDaily: UISwitch!
override func viewDidLoad() {
super.viewDidLoad()
if let pendingTrigger = pendingTrigger {
nameField.text = pendingTrigger.name
datePicker.date = pendingTrigger.fireDate
if pendingTrigger.recurrence != nil {
if pendingTrigger.recurrence.minute == 5 {
repeatSwitch.on = true
repeatDaily.enabled = false
}
if pendingTrigger.recurrence.day == 1 {
repeatDaily.on = true
repeatSwitch.enabled = false
}
} else {
repeatSwitch.on = false
repeatDaily.on = false
}
}
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
func textFieldShouldReturn(textField: UITextField!) -> Bool {
if textField == nameField {
nameField.resignFirstResponder()
}
return false
}
@IBAction func switchStateChanged(sender: UISwitch) {
if sender == repeatSwitch {
repeatDaily.on = false
repeatDaily.enabled = !sender.on
}
if sender == repeatDaily {
repeatSwitch.on = false
repeatSwitch.enabled = !sender.on
}
}
@IBAction func saveTrigger(sender: AnyObject) {
if let pendingTrigger = pendingTrigger {
let triggerName = self.nameField.text
let calendar = NSCalendar.currentCalendar()
let selectedDate = self.datePicker.date
let dateComp = calendar.components(NSCalendarUnit.CalendarUnitSecond | .CalendarUnitMinute | .CalendarUnitHour | .CalendarUnitDay | .CalendarUnitMonth | .CalendarUnitYear | .CalendarUnitEra , fromDate: selectedDate)
let fireDate = calendar.dateWithEra(dateComp.era, year: dateComp.year, month: dateComp.month, day: dateComp.day, hour: dateComp.hour, minute: dateComp.minute, second: 0, nanosecond: 0)
var recurrenceComp:NSDateComponents?
if repeatSwitch.on || repeatDaily.on {
recurrenceComp = NSDateComponents()
if repeatSwitch.on {
recurrenceComp?.minute = 5
}
if repeatDaily.on {
recurrenceComp?.day = 1
}
}
pendingTrigger.updateRecurrence(recurrenceComp) {
error in
if error != nil {
NSLog("Failed updating recurrence, error:\(error)")
}
}
pendingTrigger.updateName(triggerName) {
error in
if error != nil {
NSLog("Failed updating fire date, error:\(error)")
}
}
pendingTrigger.updateFireDate(fireDate) {
error in
if error != nil {
NSLog("Failed updating fire date, error:\(error)")
} else {
self.navigationController?.popViewControllerAnimated(true)
}
}
} else {
if let currentHome = Core.sharedInstance.currentHome {
let triggerName = self.nameField.text
let calendar = NSCalendar.currentCalendar()
let selectedDate = self.datePicker.date
let dateComp = calendar.components(NSCalendarUnit.CalendarUnitSecond | .CalendarUnitMinute | .CalendarUnitHour | .CalendarUnitDay | .CalendarUnitMonth | .CalendarUnitYear | .CalendarUnitEra , fromDate: selectedDate)
let fireDate = calendar.dateWithEra(dateComp.era, year: dateComp.year, month: dateComp.month, day: dateComp.day, hour: dateComp.hour, minute: dateComp.minute, second: 0, nanosecond: 0)
var recurrenceComp:NSDateComponents?
if repeatSwitch.on || repeatDaily.on {
recurrenceComp = NSDateComponents()
if repeatSwitch.on {
recurrenceComp?.minute = 5
}
if repeatDaily.on {
recurrenceComp?.day = 1
}
}
let trigger = HMTimerTrigger(name: triggerName, fireDate: fireDate, timeZone: nil, recurrence: recurrenceComp, recurrenceCalendar: nil)
currentHome.addTrigger(trigger) {
[weak self]
error in
if error != nil {
NSLog("Failed to add Time Trigger, Error: \(error)")
} else {
self?.navigationController?.popViewControllerAnimated(true)
}
}
}
}
}
}
| mit | da6111ad87c53acc58a03d8cd49ff862 | 38.316547 | 231 | 0.546203 | 5.553862 | false | false | false | false |
strike65/SwiftyStats | SwiftyStats/CommonSource/SpecialFunctions/Airy/Alg_838.swift | 1 | 13547 | /*
Copyright (2017-2019) strike65
GNU GPL 3+
This program is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, version 3 of the License.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with this program. If not, see <http://www.gnu.org/licenses/>.
*/
import Foundation
internal class Airy<T: SSFloatingPoint>: NSObject {
private var iflag: Int
private var iregion: Int
private var vars: AiryVariables<T>!
override init() {
vars = AiryVariables.init()
iflag = 0
iregion = 0
}
internal func airy_bir(x: T) -> (bi: T, dbi: T, error: Int) {
var nn: Int
var Pxi, Qxi, Rxi, Sxi, bi0s, bi1s, a, b: T
var bi, dbi: T
var err: Int
var tsm: Array<Array<T>> = Array<Array<T>>.init(repeating: Array<T>.init(repeating: 0, count: 2), count: 2)
iflag = 0
vars.r_global = abs(x)
vars.x_global = x
bi0s = 0
bi1s = 0
flows()
var ex1: T
var ex2: T
var ex3: T
if iflag == 0 {
vars.xi_global = T.twothirds * vars.r_global * sqrt(vars.r_global)
iregion = 0
if (vars.r_global >= vars.r_min) {
if (vars.x_global > T.zero) {
iregion = 1
}
else {
iregion = 2
}
}
else {
iregion = 3
}
switch iregion {
case 1:
bi0s = SSMath.pow1(vars.x_global, T.fourth)
bi1s = 0
a = 1 / (T.sqrtpi * bi0s)
b = bi0s / T.sqrtpi
asymp1r(F1: &bi0s, F2: &bi1s)
bi0s = a * bi0s
bi1s = b * bi1s
bi0s = SSMath.exp1(vars.xi_global) * bi0s
bi1s = SSMath.exp1(vars.xi_global) * bi1s
case 2:
bi1s = SSMath.pow1(vars.r_global, T.fourth) /* dummy storage of number */
bi0s = vars.xi_global - T.pi * T.fourth
a = 1 / (T.sqrtpi * bi1s)
b = bi1s / T.sqrtpi
Rxi = 0
Sxi = 0
Qxi = 0
Pxi = 0
asymp2r(Pxi: &Pxi, Qxi: &Qxi, Rxi: &Rxi, Sxi: &Sxi)
bi1s = vars.xi_global - T.pi * T.fourth
ex1 = -Pxi * SSMath.sin1(bi1s)
ex2 = Qxi * SSMath.cos1(bi1s)
ex3 = ex1 + ex2
bi0s = a * ex3
ex1 = Rxi * SSMath.cos1(bi1s)
ex2 = Sxi * SSMath.sin1(bi1s)
ex3 = ex1 + ex2
bi1s = b * ex3
case 3:
nn = Helpers.integerValue(ceil( Helpers.makeFP(vars.n_parts) * vars.r_global / vars.r_min))
taylorr(nn: nn, x_start: 0, tsm: &tsm)
bi0s = tsm[0][0] * bi0zer() + tsm[0][1] * bi1zer()
bi1s = tsm[1][0] * bi0zer() + tsm[1][1] * bi1zer()
default:
bi0s = T.zero
bi1s = T.zero
iflag = -100
}
}
switch iflag {
case -10..<(-1):
bi = 0
dbi = 0
err = iflag
case 0...2:
bi = bi0s
dbi = bi1s
err = 0
case 5:
bi = bi0zer()
dbi = bi1zer()
err = 0
default:
bi = 0
dbi = 0
err = 0
}
return (bi: bi, dbi: dbi, error: err)
}
internal func airy_air(x: T) -> (ai: T, dai: T, error: Int) {
var nn: Int
var Pxi, Qxi, Rxi, Sxi, ai0s, ai1s, a, b, xi0: T
var ai, dai: T
var err: Int
var tsm: Array<Array<T>> = Array<Array<T>>.init(repeating: Array<T>.init(repeating: 0, count: 2), count: 2)
iflag = 0
vars.r_global = abs(x)
vars.x_global = x
if vars.x_global < 0 {
err = -1
}
ai0s = 0
ai1s = 0
flows()
var ex1: T
var ex2: T
var ex3: T
if iflag == 0 {
vars.xi_global = T.twothirds * vars.r_global * sqrt(vars.r_global)
iregion = 0
if vars.r_global >= vars.r_min {
if vars.x_global > T.zero {
iregion = 1
}
else {
iregion = 2
}
}
else if vars.x_global > T.zero {
iregion = 3
}
else {
iregion = 4
}
switch iregion {
case 1:
ai1s = 2 * T.sqrtpi
ai0s = SSMath.pow1(vars.x_global, T.fourth)
a = 1 / (ai1s * ai0s)
b = -ai0s / ai1s
iflag = 10
asymp1r(F1: &ai0s, F2: &ai1s)
ai0s = a * ai0s
ai1s = b * ai1s
ai0s = SSMath.exp1(-vars.xi_global) * ai0s
ai1s = SSMath.exp1(-vars.xi_global) * ai1s
case 2:
ai1s = SSMath.pow1(vars.r_global, T.fourth)
a = 1 / (T.sqrtpi * ai1s)
b = ai1s / T.sqrtpi
Rxi = 0
Sxi = 0
Qxi = 0
Pxi = 0
asymp2r(Pxi: &Pxi, Qxi: &Qxi, Rxi: &Rxi, Sxi: &Sxi)
ai1s = vars.xi_global - T.pifourth
ex1 = Pxi * SSMath.cos1(ai1s)
ex2 = Qxi * SSMath.sin1(ai1s)
ex3 = ex1 + ex2
ai0s = a * ex3
ex1 = Rxi * SSMath.sin1(ai1s)
ex2 = Sxi * SSMath.cos1(ai1s)
ex3 = ex1 - ex2
ai1s = b * ex3
case 3:
ex1 = (T.one - vars.r_global / vars.r_min)
ex2 = Helpers.makeFP(vars.n_parts)
ex3 = ex2 / ex1
nn = Helpers.integerValue(ceil(ex3))
ai1s = T.twosqrtpi
ai0s = SSMath.pow1(vars.r_min, T.fourth)
a = 1 / (ai1s * ai0s)
b = -ai0s / ai1s
xi0 = T.twothirds * vars.r_min * sqrt(vars.r_min)
iflag = 10
asymp1r(F1: &ai0s, F2: &ai1s, x_in: xi0)
taylorr(nn: nn, x_start: vars.r_min, tsm: &tsm)
a = a * SSMath.exp1(-xi0) * ai0s
b = b * SSMath.exp1(-xi0) * ai1s
ai0s = tsm[0][0] * a + tsm[0][1] * b
ai1s = tsm[1][0] * a + tsm[1][1] * b
/*
if (mod_local) then
ai0s = exp(xi_global)*ai0s
ai1s = exp(xi_global)*ai1s
end if
*/
case 4:
nn = Helpers.integerValue(ceil( Helpers.makeFP(vars.n_parts) * vars.r_global / vars.r_min))
taylorr(nn: nn, x_start: T.zero, tsm: &tsm)
ai0s = tsm[0][0] * ai0zer() + tsm[0][1] * ai1zer()
ai1s = tsm[1][0] * ai0zer() + tsm[1][1] * ai1zer()
default:
ai0s = T.zero
ai1s = T.zero
iflag = -100
}
}
switch iflag {
case -10..<(-1):
ai = T.zero
dai = T.zero
err = iflag
case 0...2:
ai = ai0s
dai = ai1s
err = 0
case 5:
ai = ai0zer()
dai = ai1zer()
err = 0
default:
ai = T.zero
dai = T.zero
err = iflag
}
return (ai: ai, dai: dai, error: err)
}
private func flows() {
var tol: T
var e1, e2, e3, e4: T
if vars.r_global >= vars.r_uplimit {
iflag = -6
return
}
if vars.r_global <= vars.r_lolimit {
iflag = 5
return
}
if vars.x_global > T.zero {
e1 = -SSMath.log1(T.twosqrtpi * SSMath.pow1(vars.r_global, T.fourth)) - SSMath.log1(T.leastNormalMagnitude)
e2 = -SSMath.log1(T.twosqrtpi / SSMath.pow1(vars.r_global, T.fourth)) - SSMath.log1(T.leastNormalMagnitude)
e3 = SSMath.log1(T.twosqrtpi / SSMath.pow1(vars.r_global, T.fourth)) + SSMath.log1(T.greatestFiniteMagnitude)
e4 = SSMath.log1(T.twosqrtpi * SSMath.pow1(vars.r_global, T.fourth)) + SSMath.log1(T.greatestFiniteMagnitude)
tol = min( e1,e2,e3,e4) - 15
if vars.r_global >= ( Helpers.makeFP(1.5) * SSMath.pow1(tol, T.twothirds)) {
iflag = -7
return
}
}
}
private func asymp1r(F1: inout T, F2: inout T, x_in: T? = nil) {
var xir: T
F1 = vars.ucoef[vars.n_asymp - 1]
F2 = vars.vcoef[vars.n_asymp - 1]
if x_in != nil {
xir = 1 / x_in!
}
else {
xir = 1 / vars.xi_global
}
if iflag == 10 {
xir = -xir
}
for i in stride(from: vars.n_asymp - 2, through: 0, by: -1) {
F1 = vars.ucoef[i] + xir * F1
F2 = vars.vcoef[i] + xir * F2
}
F1 = 1 + xir * F1
F2 = 1 + xir * F2
iflag = 0
}
private func asymp2r(Pxi: inout T, Qxi: inout T, Rxi: inout T, Sxi: inout T) {
var xir: T
var itemp: Int = Helpers.integerValue(floor(T.half * Helpers.makeFP(vars.n_asymp) - T.half))
if itemp % 2 != 0 {
itemp = itemp - 1
}
Pxi = vars.ucoef[itemp - 1]
Qxi = vars.ucoef[itemp - 2]
Rxi = vars.vcoef[itemp - 1]
Sxi = vars.vcoef[itemp - 2]
xir = 1 / SSMath.pow1(vars.xi_global, 2)
for i in stride(from: itemp - 3, through: 1, by: -2) {
Pxi = (vars.ucoef[i] - xir * Pxi)
Rxi = (vars.vcoef[i] - xir * Rxi)
Qxi = (vars.ucoef[i - 1] - xir * Qxi)
Sxi = (vars.vcoef[i - 1] - xir * Sxi)
}
Pxi = 1 - xir * Pxi
Rxi = 1 - xir * Rxi
Qxi = Qxi / vars.xi_global
Sxi = Sxi / vars.xi_global
iflag = 0
}
private func taylorr(nn: Int, x_start: T, tsm: inout Array<Array<T>>) {
var ifl, jfl: T
var h, xm: T
var pterm: Array<T> = Array<T>.init(repeating: 0, count: vars.n_taylor + 1)
var qterm: Array<T> = Array<T>.init(repeating: 0, count: vars.n_taylor + 1)
var Phi: Array<Array<Array<T>>> = Array<Array<Array<T>>>.init(repeating: Array<Array<T>>.init(repeating: Array<T>.init(repeating: 0, count: 2), count: 2), count: nn)
h = (vars.x_global - x_start) / Helpers.makeFP(nn)
var ex1: T
var ex2: T
var ex3: T
for i in stride(from: 1, through: nn, by: 1) {
ifl = Helpers.makeFP(i)
xm = (x_start + h * (ifl - 1))
pterm[0] = 1
pterm[1] = T.zero
pterm[2] = xm * pterm[0] * T.half
qterm[0] = T.zero
qterm[1] = 1
qterm[2] = T.zero
for j in stride(from: 3, through: vars.n_taylor, by: 1) {
jfl = Helpers.makeFP(j)
ex1 = jfl * jfl - jfl
ex2 = xm * pterm[j - 2]
ex3 = ex2 + pterm[j - 3]
pterm[j] = ex3 / ex1
ex1 = jfl * jfl - jfl
ex2 = xm * qterm[j - 2]
ex3 = ex2 + qterm[j - 3]
qterm[j] = ex3 / ex1
}
Phi[i - 1][0][0] = pterm[vars.n_taylor]
Phi[i - 1][1][0] = pterm[vars.n_taylor] * Helpers.makeFP(vars.n_taylor)
Phi[i - 1][0][1] = qterm[vars.n_taylor]
Phi[i - 1][1][1] = qterm[vars.n_taylor] * Helpers.makeFP(vars.n_taylor)
for j in stride(from: vars.n_taylor - 1, through: 1, by: -1) {
jfl = Helpers.makeFP(j)
Phi[i - 1][0][0] = pterm[j] + h * Phi[i - 1][0][0]
Phi[i - 1][1][0] = pterm[j] * jfl + h * Phi[i - 1][1][0]
Phi[i - 1][0][1] = qterm[j] + h * Phi[i - 1][0][1]
Phi[i - 1][1][1] = qterm[j] * jfl + h * Phi[i - 1][1][1]
}
Phi[i - 1][0][0] = pterm[0] + h * Phi[i - 1][0][0]
Phi[i - 1][0][1] = qterm[0] + h * Phi[i - 1][0][1]
}
for i in stride(from: 0, to: nn - 1, by: 1) {
Phi[i + 1] = matmul(matA: Phi[i + 1], aRows: 2, aCols: 2, matB: Phi[i], bRows: 2, bCols: 2)
}
tsm = Phi.last!
}
private func matmul<T: FloatingPoint>(matA: Array<Array<T>>, aRows m: Int, aCols n: Int, matB: Array<Array<T>>, bRows p: Int, bCols q: Int) -> Array<Array<T>> {
if n != p {
fatalError()
}
var sum: T = 0
var C: Array<Array<T>> = Array<Array<T>>.init(repeating: Array<T>.init(repeating: 0, count: q), count: m)
for c in stride(from: 0, to: m, by: 1) {
for d in stride(from: 0, to: q, by: 1) {
for k in stride(from: 0, to: p, by: 1) {
sum = sum + matA[c][k] * matB[k][d]
}
C[c][d] = sum
sum = 0
}
}
return C
}
}
| gpl-3.0 | a95df79696972b3413c3c7ce75c9c906 | 34.370757 | 173 | 0.433971 | 3.049752 | false | false | false | false |
xwu/swift | test/Concurrency/Runtime/async_task_locals_wrapper.swift | 1 | 2424 | // RUN: %target-run-simple-swift( -Xfrontend -disable-availability-checking -parse-as-library %import-libdispatch) | %FileCheck %s
// REQUIRES: executable_test
// REQUIRES: concurrency
// REQUIRES: libdispatch
// rdar://76038845
// REQUIRES: concurrency_runtime
// UNSUPPORTED: back_deployment_runtime
@available(SwiftStdlib 5.5, *)
enum TL {
@TaskLocal
static var number: Int = 0
}
@available(SwiftStdlib 5.5, *)
@discardableResult
func printTaskLocal<V>(
_ key: TaskLocal<V>,
_ expected: V? = nil,
file: String = #file, line: UInt = #line
) -> V? {
let value = key.get()
print("\(key) (\(value)) at \(file):\(line)")
if let expected = expected {
assert("\(expected)" == "\(value)",
"Expected [\(expected)] but found: \(value), at \(file):\(line)")
}
return expected
}
// ==== ------------------------------------------------------------------------
@available(SwiftStdlib 5.5, *)
func async_let_nested() async {
print("TL: \(TL.$number)")
_ = printTaskLocal(TL.$number) // CHECK: TaskLocal<Int>(defaultValue: 0) (0)
async let x1: () = TL.$number.withValue(2) {
async let x2 = printTaskLocal(TL.$number) // CHECK: TaskLocal<Int>(defaultValue: 0) (2)
@Sendable
func test() async {
printTaskLocal(TL.$number) // CHECK: TaskLocal<Int>(defaultValue: 0) (2)
async let x31 = printTaskLocal(TL.$number) // CHECK: TaskLocal<Int>(defaultValue: 0) (2)
_ = await x31
}
async let x3: () = test()
_ = await x2
await x3
}
_ = await x1
printTaskLocal(TL.$number) // CHECK: TaskLocal<Int>(defaultValue: 0) (0)
}
@available(SwiftStdlib 5.5, *)
func async_let_nested_skip_optimization() async {
async let x1: Int? = TL.$number.withValue(2) {
async let x2: Int? = { () async -> Int? in
async let x3: Int? = { () async -> Int? in
async let x4: Int? = { () async -> Int? in
async let x5: Int? = { () async -> Int? in
// assert(TL.$number == 2)
async let xx = printTaskLocal(TL.$number) // CHECK: TaskLocal<Int>(defaultValue: 0) (2)
return await xx
}()
return await x5
}()
return await x4
}()
return await x3
}()
return await x2
}
_ = await x1
}
@available(SwiftStdlib 5.5, *)
@main struct Main {
static func main() async {
await async_let_nested()
await async_let_nested_skip_optimization()
}
}
| apache-2.0 | b6a306a95c8d453b632d911da192ecb6 | 26.545455 | 130 | 0.580446 | 3.596439 | false | false | false | false |
Tomikes/eidolon | Kiosk/Bid Fulfillment/ConfirmYourBidArtsyLoginViewController.swift | 6 | 6954 | import UIKit
import Moya
import ReactiveCocoa
import Swift_RAC_Macros
class ConfirmYourBidArtsyLoginViewController: UIViewController {
@IBOutlet var emailTextField: UITextField!
@IBOutlet var passwordTextField: TextField!
@IBOutlet var bidDetailsPreviewView: BidDetailsPreviewView!
@IBOutlet var useArtsyBidderButton: UIButton!
@IBOutlet var confirmCredentialsButton: Button!
var createNewAccount = false
lazy var provider:ReactiveCocoaMoyaProvider<ArtsyAPI> = Provider.sharedProvider
class func instantiateFromStoryboard(storyboard: UIStoryboard) -> ConfirmYourBidArtsyLoginViewController {
return storyboard.viewControllerWithID(.ConfirmYourBidArtsyLogin) as! ConfirmYourBidArtsyLoginViewController
}
override func viewDidLoad() {
super.viewDidLoad()
let titleString = useArtsyBidderButton.titleForState(useArtsyBidderButton.state)! ?? ""
let attributes = [NSUnderlineStyleAttributeName: NSUnderlineStyle.StyleSingle.rawValue,
NSFontAttributeName: useArtsyBidderButton.titleLabel!.font];
let attrTitle = NSAttributedString(string: titleString, attributes:attributes)
useArtsyBidderButton.setAttributedTitle(attrTitle, forState:useArtsyBidderButton.state)
let nav = self.fulfillmentNav()
bidDetailsPreviewView.bidDetails = nav.bidDetails
emailTextField.text = nav.bidDetails.newUser.email ?? ""
let emailTextSignal = emailTextField.rac_textSignal()
let passwordTextSignal = passwordTextField.rac_textSignal()
RAC(nav.bidDetails.newUser, "email") <~ emailTextSignal.takeUntil(viewWillDisappearSignal())
RAC(nav.bidDetails.newUser, "password") <~ passwordTextSignal.takeUntil(viewWillDisappearSignal())
let inputIsEmail = emailTextSignal.map(stringIsEmailAddress)
let passwordIsLongEnough = passwordTextSignal.map(isZeroLengthString).not()
let formIsValid = RACSignal.combineLatest([inputIsEmail, passwordIsLongEnough]).and()
confirmCredentialsButton.rac_command = RACCommand(enabled: formIsValid) { [weak self] _ in
if (self == nil) {
return RACSignal.empty()
}
return self!.xAuthSignal().`try` { (accessTokenDict, errorPointer) -> Bool in
if let accessToken = accessTokenDict["access_token"] as? String {
self?.fulfillmentNav().xAccessToken = accessToken
return true
} else {
errorPointer.memory = NSError(domain: "eidolon", code: 123, userInfo: [NSLocalizedDescriptionKey : "Error fetching access_token"])
return false
}
}.then {
return self?.fulfillmentNav().updateUserCredentials() ?? RACSignal.empty()
}.then {
return self?.creditCardSignal().doNext { (cards) -> Void in
if (self == nil) { return }
if cards.count > 0 {
self!.performSegue(.EmailLoginConfirmedHighestBidder)
} else {
self!.performSegue(.ArtsyUserHasNotRegisteredCard)
}
} ?? RACSignal.empty()
}.doError { [weak self] (error) -> Void in
logger.log("Error logging in: \(error.localizedDescription)")
logger.log("Error Logging in, likely bad auth creds, email = \(self?.emailTextField.text)")
self?.showAuthenticationError()
}
}
}
override func viewWillAppear(animated: Bool) {
super.viewWillAppear(animated)
if emailTextField.text.isNilOrEmpty {
emailTextField.becomeFirstResponder()
} else {
passwordTextField.becomeFirstResponder()
}
}
func showAuthenticationError() {
confirmCredentialsButton.flashError("Wrong login info")
passwordTextField.flashForError()
fulfillmentNav().bidDetails.newUser.password = ""
passwordTextField.text = ""
}
func xAuthSignal() -> RACSignal {
let endpoint: ArtsyAPI = ArtsyAPI.XAuth(email: emailTextField.text ?? "", password: passwordTextField.text ?? "")
return provider.request(endpoint).filterSuccessfulStatusCodes().mapJSON()
}
@IBAction func forgotPasswordTapped(sender: AnyObject) {
let alertController = UIAlertController(title: "Forgot Password", message: "Please enter your email address and we'll send you a reset link.", preferredStyle: .Alert)
let submitAction = UIAlertAction(title: "Send", style: .Default) { [weak alertController] (_) in
let emailTextField = alertController!.textFields![0]
self.sendForgotPasswordRequest(emailTextField.text ?? "")
return
}
submitAction.enabled = false
submitAction.enabled = stringIsEmailAddress(emailTextField.text).boolValue
let cancelAction = UIAlertAction(title: "Cancel", style: .Cancel) { (_) in }
alertController.addTextFieldWithConfigurationHandler { (textField) in
textField.placeholder = "[email protected]"
textField.text = self.emailTextField.text
NSNotificationCenter.defaultCenter().addObserverForName(UITextFieldTextDidChangeNotification, object: textField, queue: NSOperationQueue.mainQueue()) { (notification) in
submitAction.enabled = stringIsEmailAddress(textField.text).boolValue
}
}
alertController.addAction(submitAction)
alertController.addAction(cancelAction)
self.presentViewController(alertController, animated: true) {}
}
func sendForgotPasswordRequest(email: String) {
let endpoint: ArtsyAPI = ArtsyAPI.LostPasswordNotification(email: email)
XAppRequest(endpoint).filterSuccessfulStatusCodes().subscribeNext { (json) -> Void in
logger.log("Sent forgot password request")
}
}
func creditCardSignal() -> RACSignal {
let endpoint: ArtsyAPI = ArtsyAPI.MyCreditCards
let authProvider = self.fulfillmentNav().loggedInProvider!
return authProvider.request(endpoint).filterSuccessfulStatusCodes().mapJSON().mapToObjectArray(Card.self)
}
@IBAction func useBidderTapped(sender: AnyObject) {
for controller in navigationController!.viewControllers {
if controller.isKindOfClass(ConfirmYourBidViewController.self) {
navigationController!.popToViewController(controller, animated:true);
break;
}
}
}
}
private extension ConfirmYourBidArtsyLoginViewController {
@IBAction func dev_hasCardTapped(sender: AnyObject) {
self.performSegue(.EmailLoginConfirmedHighestBidder)
}
@IBAction func dev_noCardFoundTapped(sender: AnyObject) {
self.performSegue(.ArtsyUserHasNotRegisteredCard)
}
}
| mit | 734d31ddbac4d14470552802919f50c3 | 41.925926 | 181 | 0.671268 | 5.603546 | false | false | false | false |
LYM-mg/MGDS_Swift | MGDS_Swift/MGDS_Swift/Class/Home/Tencent/TencentCell.swift | 1 | 2281 | //
// TencentCell.swift
// MGDS_Swift
//
// Created by i-Techsys.com on 17/1/18.
// Copyright © 2017年 i-Techsys. All rights reserved.
//
import UIKit
import Kingfisher
@objc
protocol TencentCellProtocol: NSObjectProtocol{
@objc func playBtnClick(cell: TencentCell,model: VideoList)
}
class TencentCell: UITableViewCell {
// MARK: - 属性
@IBOutlet weak var titleLabel: UILabel!
@IBOutlet weak var descriptionLabel: UILabel! // 视频描述
@IBOutlet weak var playImageV: UIImageView! // 播放背景
@IBOutlet weak var timeDurationLabel: UILabel! // 视频时长
@IBOutlet weak var countLabel: UILabel! // 播放按钮
weak var delegate: TencentCellProtocol?
lazy var playBtn: UIButton = UIButton(frame: CGRect(x: 0, y: 0, width: 60, height: 60))
var model: VideoList? {
didSet {
self.titleLabel?.text = model?.title
self.descriptionLabel.text = model?.topicDesc
self.playImageV.kf.setImage(with: URL(string: (model?.cover)!)!, placeholder: UIImage(named: "10"))
// length代替playCount,因为playCount都是0不好看
self.countLabel.text = "播放: \(model!.length!)次"
self.timeDurationLabel.text = (model!.ptime! as NSString).substring(with: NSRange(location: 12, length: 4))
}
}
@objc func playBtnClick(_ sender: UIButton) {
if (delegate?.responds(to: #selector(TencentCellProtocol.playBtnClick)))! {
delegate?.playBtnClick(cell: self, model: model!)
}
}
// MARK: - 系统方法
override func awakeFromNib() {
super.awakeFromNib()
// 代码添加playerBtn到imageView上
self.playBtn.setImage(UIImage(named: "video_play_btn_bg")!, for: .normal)
self.playBtn.addTarget(self, action: #selector(self.playBtnClick(_:)), for: .touchUpInside)
self.playImageV.addSubview(self.playBtn)
}
override func setSelected(_ selected: Bool, animated: Bool) {
super.setSelected(selected, animated: animated)
}
override func layoutSubviews() {
super.layoutSubviews()
self.playBtn.center = CGPoint(x: MGScreenW/2, y: playImageV.frame.size.height/2)
}
}
| mit | c16c8efb2fc49ad81d176f81ebd8a61f | 33.3125 | 119 | 0.639344 | 4.021978 | false | false | false | false |
chrisamanse/CryptoKit | Sources/CryptoKit/Hash Algorithms/Implementations/MD5.swift | 1 | 4314 | //
// MD5.swift
// CryptoKit
//
// Created by Chris Amanse on 28/08/2016.
//
//
import Foundation
public enum MD5: HashAlgorithm {
public static var outputSize: UInt {
return 16
}
public static var blockSize: UInt {
return 64
}
}
extension MD5: MerkleDamgardConstructor {
public static var initializationVector: [UInt32] {
return [0x67452301, 0xefcdab89, 0x98badcfe, 0x10325476]
}
public static var rounds: UInt {
return 64
}
public static func compress(_ data: Data) -> [UInt32] {
// Initialize hash value
var h = self.initializationVector
// Divide into 512-bit (64-byte) chunks
// Since data length is 0 bytes (mod 64), all chunks are 64 bytes
let chunkLength = Int(self.blockSize)
for index in stride(from: data.startIndex, to: data.endIndex, by: chunkLength) {
// Get 512-bit chunk
let chunk = data.subdata(in: index ..< index + chunkLength)
// Divide chunk into 32-bit words (512 is divisible by 32, thus all words are 32 bits)
// Since 512 is divisible by 32, simply create array by converting the Data pointer to a UInt32 array pointer
let M = chunk.withUnsafeBytes { (ptr: UnsafePointer<UInt32>) -> [UInt32] in
// 512 / 32 = 16 words
return Array(UnsafeBufferPointer(start: ptr, count: 16))
}
// Initiaize hash value for this chunk
var A = h[0]
var B = h[1]
var C = h[2]
var D = h[3]
// Main loop
for i in 0 ..< Int(self.rounds) {
// Divide 0..<64 into four rounds, i.e. 0..<16 = round 1, 16..<32 = round2, etc.
let round = i / 16
// Calculate F and g depending on round
let F: UInt32
let g: Int
switch round {
case 0:
F = (B & C) | ((~B) & D)
g = i
case 1:
F = (D & B) | ((~D) & C)
g = ((5 &* i) &+ 1) % 16
case 2:
F = B ^ C ^ D
g = ((3 &* i) &+ 5) % 16
case 3:
F = C ^ (B | (~D))
g = (7 &* i) % 16
default:
F = 0
g = 0
}
// Swap values
let newB = B &+ ((A &+ F &+ K(i) &+ M[g])) <<< s(i)
let oldD = D
D = C
C = B
B = newB
A = oldD
}
// Add current chunk's hash to result (allow overflow)
let currentHash = [A, B, C, D]
for i in 0..<h.count {
h[i] = h[i] &+ currentHash[i]
}
}
return h
}
}
extension MD5 {
/// Get binary integer part of the sines of integers (Radians)
static func K(_ i: Int) -> UInt32 {
return UInt32(floor(4294967296 * abs(sin(Double(i) + 1))))
}
/// Get per-round shift amounts
static func s(_ i: Int) -> UInt32 {
let row = i / 16
let column = i % 4
switch row {
case 0:
switch column {
case 0: return 7
case 1: return 12
case 2: return 17
case 3: return 22
default: return 0
}
case 1:
switch column {
case 0: return 5
case 1: return 9
case 2: return 14
case 3: return 20
default: return 0
}
case 2:
switch column {
case 0: return 4
case 1: return 11
case 2: return 16
case 3: return 23
default: return 0
}
case 3:
switch column {
case 0: return 6
case 1: return 10
case 2: return 15
case 3: return 21
default: return 0
}
default:
return 0
}
}
}
| mit | 37c95fe7949d49082726a64338b7e846 | 27.76 | 121 | 0.424432 | 4.456612 | false | false | false | false |
lhc70000/iina | iina/ISO639TokenFieldDelegate.swift | 2 | 2276 | //
// ISO639TokenField.swift
// iina
//
// Created by Collider LI on 23/9/2018.
// Copyright © 2018 lhc. All rights reserved.
//
import Cocoa
class ISO639TokenFieldDelegate: NSObject, NSTokenFieldDelegate {
class Token: NSObject {
var name: String
init(_ name: String) {
self.name = name
}
}
func tokenField(_ tokenField: NSTokenField, styleForRepresentedObject representedObject: Any) -> NSTokenField.TokenStyle {
return .rounded
}
func tokenField(_ tokenField: NSTokenField, hasMenuForRepresentedObject representedObject: Any) -> Bool {
return false
}
func tokenField(_ tokenField: NSTokenField, completionsForSubstring substring: String, indexOfToken tokenIndex: Int, indexOfSelectedItem selectedIndex: UnsafeMutablePointer<Int>?) -> [Any]? {
let lowSubString = substring.lowercased()
let matches = ISO639Helper.languages.filter { lang in
return lang.name.reduce(false) { $1.lowercased().hasPrefix(lowSubString) || $0 }
}
return matches.map { $0.description }
}
func tokenField(_ tokenField: NSTokenField, representedObjectForEditing editingString: String) -> Any? {
if let code = Regex.iso639_2Desc.captures(in: editingString)[at: 1] {
return Token(code)
} else {
return Token(editingString)
}
}
func tokenField(_ tokenField: NSTokenField, displayStringForRepresentedObject representedObject: Any) -> String? {
if let token = representedObject as? Token {
return token.name
} else {
return representedObject as? String
}
}
}
@objc(ISO639Transformer) class ISO639Transformer: ValueTransformer {
static override func allowsReverseTransformation() -> Bool {
return true
}
static override func transformedValueClass() -> AnyClass {
return NSString.self
}
override func transformedValue(_ value: Any?) -> Any? {
guard let str = value as? NSString else { return nil }
if str.length == 0 { return [] }
return str.components(separatedBy: ",").map { ISO639TokenFieldDelegate.Token($0) }
}
override func reverseTransformedValue(_ value: Any?) -> Any? {
guard let arr = value as? NSArray else { return "" }
return arr.map{ ($0 as! ISO639TokenFieldDelegate.Token).name }.joined(separator: ",")
}
}
| gpl-3.0 | 9ff298515dcd8c2c9df678f5ea26cc79 | 28.545455 | 193 | 0.698462 | 4.408915 | false | false | false | false |
Shopify/mobile-buy-sdk-ios | Buy/Generated/Storefront/CheckoutLineItemsAddPayload.swift | 1 | 5694 | //
// CheckoutLineItemsAddPayload.swift
// Buy
//
// Created by Shopify.
// Copyright (c) 2017 Shopify Inc. All rights reserved.
//
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in
// all copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
// THE SOFTWARE.
//
import Foundation
extension Storefront {
/// Return type for `checkoutLineItemsAdd` mutation.
open class CheckoutLineItemsAddPayloadQuery: GraphQL.AbstractQuery, GraphQLQuery {
public typealias Response = CheckoutLineItemsAddPayload
/// The updated checkout object.
@discardableResult
open func checkout(alias: String? = nil, _ subfields: (CheckoutQuery) -> Void) -> CheckoutLineItemsAddPayloadQuery {
let subquery = CheckoutQuery()
subfields(subquery)
addField(field: "checkout", aliasSuffix: alias, subfields: subquery)
return self
}
/// The list of errors that occurred from executing the mutation.
@discardableResult
open func checkoutUserErrors(alias: String? = nil, _ subfields: (CheckoutUserErrorQuery) -> Void) -> CheckoutLineItemsAddPayloadQuery {
let subquery = CheckoutUserErrorQuery()
subfields(subquery)
addField(field: "checkoutUserErrors", aliasSuffix: alias, subfields: subquery)
return self
}
/// The list of errors that occurred from executing the mutation.
@available(*, deprecated, message:"Use `checkoutUserErrors` instead.")
@discardableResult
open func userErrors(alias: String? = nil, _ subfields: (UserErrorQuery) -> Void) -> CheckoutLineItemsAddPayloadQuery {
let subquery = UserErrorQuery()
subfields(subquery)
addField(field: "userErrors", aliasSuffix: alias, subfields: subquery)
return self
}
}
/// Return type for `checkoutLineItemsAdd` mutation.
open class CheckoutLineItemsAddPayload: GraphQL.AbstractResponse, GraphQLObject {
public typealias Query = CheckoutLineItemsAddPayloadQuery
internal override func deserializeValue(fieldName: String, value: Any) throws -> Any? {
let fieldValue = value
switch fieldName {
case "checkout":
if value is NSNull { return nil }
guard let value = value as? [String: Any] else {
throw SchemaViolationError(type: CheckoutLineItemsAddPayload.self, field: fieldName, value: fieldValue)
}
return try Checkout(fields: value)
case "checkoutUserErrors":
guard let value = value as? [[String: Any]] else {
throw SchemaViolationError(type: CheckoutLineItemsAddPayload.self, field: fieldName, value: fieldValue)
}
return try value.map { return try CheckoutUserError(fields: $0) }
case "userErrors":
guard let value = value as? [[String: Any]] else {
throw SchemaViolationError(type: CheckoutLineItemsAddPayload.self, field: fieldName, value: fieldValue)
}
return try value.map { return try UserError(fields: $0) }
default:
throw SchemaViolationError(type: CheckoutLineItemsAddPayload.self, field: fieldName, value: fieldValue)
}
}
/// The updated checkout object.
open var checkout: Storefront.Checkout? {
return internalGetCheckout()
}
func internalGetCheckout(alias: String? = nil) -> Storefront.Checkout? {
return field(field: "checkout", aliasSuffix: alias) as! Storefront.Checkout?
}
/// The list of errors that occurred from executing the mutation.
open var checkoutUserErrors: [Storefront.CheckoutUserError] {
return internalGetCheckoutUserErrors()
}
func internalGetCheckoutUserErrors(alias: String? = nil) -> [Storefront.CheckoutUserError] {
return field(field: "checkoutUserErrors", aliasSuffix: alias) as! [Storefront.CheckoutUserError]
}
/// The list of errors that occurred from executing the mutation.
@available(*, deprecated, message:"Use `checkoutUserErrors` instead.")
open var userErrors: [Storefront.UserError] {
return internalGetUserErrors()
}
func internalGetUserErrors(alias: String? = nil) -> [Storefront.UserError] {
return field(field: "userErrors", aliasSuffix: alias) as! [Storefront.UserError]
}
internal override func childResponseObjectMap() -> [GraphQL.AbstractResponse] {
var response: [GraphQL.AbstractResponse] = []
objectMap.keys.forEach {
switch($0) {
case "checkout":
if let value = internalGetCheckout() {
response.append(value)
response.append(contentsOf: value.childResponseObjectMap())
}
case "checkoutUserErrors":
internalGetCheckoutUserErrors().forEach {
response.append($0)
response.append(contentsOf: $0.childResponseObjectMap())
}
case "userErrors":
internalGetUserErrors().forEach {
response.append($0)
response.append(contentsOf: $0.childResponseObjectMap())
}
default:
break
}
}
return response
}
}
}
| mit | 4971c4018ab49e2a63bcc384504bbcc3 | 35.974026 | 137 | 0.729715 | 4.217778 | false | false | false | false |
heptal/kathy | Kathy/IRCEntities.swift | 1 | 867 | //
// IRCEntities.swift
// Kathy
//
// Created by Michael Bujol on 6/19/16.
// Copyright © 2016 heptal. All rights reserved.
//
import Cocoa
class Channel: NSObject {
let name: String
var log: [String] = []
var users: Set<User> = []
init(name: String) {
self.name = name
}
}
class User: NSObject {
var name: String = ""
var mode: String = ""
init(_ modeName: String) {
if let matches = "([~&@%+]?)(.*)".captures(modeName), let modePart = matches.first, let namePart = matches.last {
name = namePart
mode = modePart
}
}
override var description: String {
return mode + name
}
override var hashValue: Int {
return name.hashValue
}
override func isEqual(_ object: Any?) -> Bool {
return (object as? User)?.name == name
}
}
| apache-2.0 | a34d79112bc36b7cc98be7a3c42cabe9 | 18.244444 | 121 | 0.556582 | 3.669492 | false | false | false | false |
S2dentik/Taylor | TaylorFrameworkTests/CapriceTests/Mocks/MockMessageProcessor.swift | 4 | 1882 | //
// MockMessageProcessor.swift
// Caprices
//
// Created by Dmitrii Celpan on 9/7/15.
// Copyright © 2015 yopeso.dmitriicelpan. All rights reserved.
//
import Foundation
import ExcludesFileReader
@testable import TaylorFramework
class MockMessageProcessor : MessageProcessor {
override func defaultResultDictionary() -> [String : [String]] {
var defaultDictionary = defaultDictionaryWithPathAndType()
setDefaultExcludesIfExistsToDictionary(&defaultDictionary)
return defaultDictionary
}
override func setDefaultExcludesIfExistsToDictionary(_ dictionary: inout Options) {
let fileManager = MockFileManager()
let pathToDefaultExcludesFile = fileManager.testFile("excludes", fileType: "yml")
let excludesFileReader = ExcludesFileReader()
var excludePaths = [String]()
do {
let pathToExcludesFile = pathToDefaultExcludesFile.absolutePath()
excludePaths = try excludesFileReader.absolutePathsFromExcludesFile(pathToExcludesFile, forAnalyzePath: dictionary[ResultDictionaryPathKey]![0])
} catch {
return
}
if !excludePaths.isEmpty {
dictionary[ResultDictionaryExcludesKey] = excludePaths
}
}
override func processMultipleArguments(_ arguments:[String]) -> Options {
if arguments.count.isOdd {
let optionsProcessor = MockOptionsProcessor()
return try! optionsProcessor.processOptions(arguments: arguments) // We want this to crash here if arguments are invalid
} else if arguments.containFlags {
FlagBuilder().flag(arguments.second!).execute()
return [FlagKey : [FlagKeyValue]]
} else {
print("\nInvalid options was indicated")
return Options()
}
}
}
| mit | 0cd1f77ae18122b5266d3e81cae1ebc9 | 33.2 | 156 | 0.663477 | 5.153425 | false | false | false | false |
davidozhang/spycodes | Spycodes/ViewControllers/Main/SCMainSettingsViewController.swift | 1 | 11585 | import UIKit
protocol SCMainSettingsViewControllerDelegate: class {
func mainSettings(onToggleViewCellChanged toggleViewCell: SCToggleViewCell,
settingType: SCLocalSettingType)
}
class SCMainSettingsViewController: SCModalViewController {
weak var delegate: SCMainSettingsViewControllerDelegate?
fileprivate enum Section: Int {
case about = 0
case customize = 1
case more = 2
static var count: Int {
var count = 0
while let _ = Section(rawValue: count) {
count += 1
}
return count
}
}
fileprivate enum CustomSetting: Int {
case nightMode = 0
case accessibility = 1
static var count: Int {
var count = 0
while let _ = CustomSetting(rawValue: count) {
count += 1
}
return count
}
}
fileprivate enum Link: Int {
case support = 0
case github = 1
case icons8 = 2
static var count: Int {
var count = 0
while let _ = Link(rawValue: count) {
count += 1
}
return count
}
}
fileprivate let sectionLabels: [Section: String] = [
.customize: SCStrings.section.customize.rawLocalized,
.about: SCStrings.section.about.rawLocalized,
.more: SCStrings.section.more.rawLocalized,
]
fileprivate let customizeLabels: [CustomSetting: String] = [
.nightMode: SCStrings.primaryLabel.nightMode.rawLocalized,
.accessibility: SCStrings.primaryLabel.accessibility.rawLocalized,
]
fileprivate let customizeSecondaryLabels: [CustomSetting: String] = [
.nightMode: SCStrings.secondaryLabel.nightMode.rawLocalized,
.accessibility: SCStrings.secondaryLabel.accessibility.rawLocalized,
]
fileprivate let disclosureLabels: [Link: String] = [
.support: SCStrings.primaryLabel.support.rawLocalized,
.github: SCStrings.primaryLabel.github.rawLocalized,
.icons8: SCStrings.primaryLabel.icons8.rawLocalized,
]
fileprivate var scrolled = false
@IBOutlet weak var tableView: UITableView!
@IBOutlet weak var tableViewBottomSpaceConstraint: NSLayoutConstraint!
@IBOutlet weak var tableViewLeadingSpaceConstraint: NSLayoutConstraint!
@IBOutlet weak var tableViewTrailingSpaceConstraint: NSLayoutConstraint!
@IBOutlet weak var upArrowView: UIImageView!
// MARK: Lifecycle
override func viewDidLoad() {
super.viewDidLoad()
self.uniqueIdentifier = SCConstants.viewControllers.mainSettingsViewController.rawValue
self.tableView.rowHeight = UITableViewAutomaticDimension
self.tableView.estimatedRowHeight = 44.0
self.registerTableViewCells()
}
override func viewWillAppear(_ animated: Bool) {
super.viewWillAppear(animated)
self.tableView.dataSource = self
self.tableView.delegate = self
self.tableViewBottomSpaceConstraint.constant = SCViewController.tableViewMargin
self.tableViewLeadingSpaceConstraint.constant = SCViewController.tableViewMargin
self.tableViewTrailingSpaceConstraint.constant = SCViewController.tableViewMargin
self.tableView.layoutIfNeeded()
if self.tableView.contentSize.height <= self.tableView.bounds.height {
self.upArrowView.isHidden = true
}
}
override func viewDidDisappear(_ animated: Bool) {
super.viewDidDisappear(animated)
self.tableView.dataSource = nil
self.tableView.delegate = nil
}
override func onDismissal() {
if self.tableView.contentOffset.y > 0 {
return
}
super.onDismissal()
}
fileprivate func registerTableViewCells() {
let multilineToggleViewCellNib = UINib(nibName: SCConstants.nibs.multilineToggleViewCell.rawValue, bundle: nil)
self.tableView.register(
multilineToggleViewCellNib,
forCellReuseIdentifier: SCConstants.reuseIdentifiers.nightModeToggleViewCell.rawValue
)
self.tableView.register(
multilineToggleViewCellNib,
forCellReuseIdentifier: SCConstants.reuseIdentifiers.accessibilityToggleViewCell.rawValue
)
}
}
// _____ _ _
// | ____|_ _| |_ ___ _ __ ___(_) ___ _ __ ___
// | _| \ \/ / __/ _ \ '_ \/ __| |/ _ \| '_ \/ __|
// | |___ > <| || __/ | | \__ \ | (_) | | | \__ \
// |_____/_/\_\\__\___|_| |_|___/_|\___/|_| |_|___/
// MARK: UITableViewDelegate, UITableViewDataSource
extension SCMainSettingsViewController: UITableViewDelegate, UITableViewDataSource {
func numberOfSections(in tableView: UITableView) -> Int {
return Section.count
}
func tableView(_ tableView: UITableView,
heightForHeaderInSection section: Int) -> CGFloat {
return 44.0
}
func tableView(_ tableView: UITableView,
viewForHeaderInSection section: Int) -> UIView? {
guard let sectionHeader = self.tableView.dequeueReusableCell(
withIdentifier: SCConstants.reuseIdentifiers.sectionHeaderCell.rawValue
) as? SCSectionHeaderViewCell else {
return nil
}
if let section = Section(rawValue: section) {
sectionHeader.primaryLabel.text = sectionLabels[section]
}
if self.scrolled {
sectionHeader.showBlurBackground()
} else {
sectionHeader.hideBlurBackground()
}
return sectionHeader
}
func tableView(_ tableView: UITableView,
numberOfRowsInSection section: Int) -> Int {
switch section {
case Section.customize.rawValue:
return CustomSetting.count
case Section.about.rawValue:
return 1
case Section.more.rawValue:
return Link.count
default:
return 0
}
}
func tableView(_ tableView: UITableView,
cellForRowAt indexPath: IndexPath) -> UITableViewCell {
switch indexPath.section {
case Section.customize.rawValue:
switch indexPath.row {
case CustomSetting.nightMode.rawValue:
guard let cell = self.tableView.dequeueReusableCell(
withIdentifier: SCConstants.reuseIdentifiers.nightModeToggleViewCell.rawValue
) as? SCToggleViewCell else {
return SCTableViewCell()
}
cell.synchronizeToggle()
cell.primaryLabel.text = self.customizeLabels[.nightMode]
cell.secondaryLabel.text = self.customizeSecondaryLabels[.nightMode]
cell.delegate = self
return cell
case CustomSetting.accessibility.rawValue:
guard let cell = self.tableView.dequeueReusableCell(
withIdentifier: SCConstants.reuseIdentifiers.accessibilityToggleViewCell.rawValue
) as? SCToggleViewCell else {
return SCTableViewCell()
}
cell.synchronizeToggle()
cell.primaryLabel.text = self.customizeLabels[.accessibility]
cell.secondaryLabel.text = self.customizeSecondaryLabels[.accessibility]
cell.delegate = self
return cell
default:
return SCTableViewCell()
}
case Section.about.rawValue:
guard let cell = self.tableView.dequeueReusableCell(
withIdentifier: SCConstants.reuseIdentifiers.versionViewCell.rawValue
) as? SCTableViewCell else {
return SCTableViewCell()
}
let attributedString = NSMutableAttributedString(
string: SCAppInfoManager.appVersion + " (\(SCAppInfoManager.buildNumber))"
)
attributedString.addAttribute(
NSFontAttributeName,
value: SCFonts.intermediateSizeFont(.medium) ?? 0,
range: NSMakeRange(
SCAppInfoManager.appVersion.count + 1,
SCAppInfoManager.buildNumber.count + 2
)
)
cell.primaryLabel.text = SCStrings.primaryLabel.version.rawLocalized
cell.rightLabel.attributedText = attributedString
return cell
case Section.more.rawValue:
guard let cell = self.tableView.dequeueReusableCell(
withIdentifier: SCConstants.reuseIdentifiers.disclosureViewCell.rawValue
) as? SCDisclosureViewCell else {
return SCTableViewCell()
}
if let link = Link(rawValue: indexPath.row) {
cell.primaryLabel.text = self.disclosureLabels[link]
}
return cell
default:
return SCTableViewCell()
}
}
func tableView(_ tableView: UITableView,
didSelectRowAt indexPath: IndexPath) {
self.tableView.deselectRow(at: indexPath, animated: false)
switch indexPath.section {
case Section.more.rawValue:
switch indexPath.row {
case Link.support.rawValue:
if let supportURL = URL(string: SCConstants.url.support.rawValue) {
UIApplication.shared.openURL(supportURL)
}
case Link.github.rawValue:
if let githubURL = URL(string: SCConstants.url.github.rawValue) {
UIApplication.shared.openURL(githubURL)
}
case Link.icons8.rawValue:
if let icons8URL = URL(string: SCConstants.url.icons8.rawValue) {
UIApplication.shared.openURL(icons8URL)
}
default:
return
}
default:
return
}
}
func scrollViewDidScroll(_ scrollView: UIScrollView) {
if self.tableView.contentOffset.y <= 0 {
self.upArrowView.isHidden = false
} else {
self.upArrowView.isHidden = true
}
if self.tableView.contentOffset.y > 0 {
if self.scrolled {
return
}
self.scrolled = true
self.disableSwipeGestureRecognizer()
} else {
if !self.scrolled {
return
}
self.scrolled = false
self.enableSwipeGestureRecognizer()
}
self.tableView.reloadData()
}
}
// MARK: SCToggleViewCellDelegate
extension SCMainSettingsViewController: SCToggleViewCellDelegate {
func toggleViewCell(onToggleViewCellChanged cell: SCToggleViewCell, enabled: Bool) {
if let reuseIdentifier = cell.reuseIdentifier {
switch reuseIdentifier {
case SCConstants.reuseIdentifiers.nightModeToggleViewCell.rawValue:
SCLocalStorageManager.instance.enableLocalSetting(.nightMode, enabled: enabled)
super.updateModalAppearance()
self.tableView.reloadData()
self.delegate?.mainSettings(onToggleViewCellChanged: cell, settingType: .nightMode)
case SCConstants.reuseIdentifiers.accessibilityToggleViewCell.rawValue:
SCLocalStorageManager.instance.enableLocalSetting(.accessibility, enabled: enabled)
default:
break
}
}
}
}
| mit | fe3017eab9d31a27607277a9f38da031 | 33.58209 | 119 | 0.604661 | 5.446638 | false | false | false | false |
git-hushuai/MOMO | MMHSMeterialProject/UINavigationController/BusinessFile/ModelAnalysis/Reflect/Dict2Model/Reflect+Parse.swift | 1 | 9253 | //
// Reflect+Parse.swift
// Reflect
//
// Created by 成林 on 15/8/23.
// Copyright (c) 2015年 冯成林. All rights reserved.
//
import Foundation
extension Reflect{
class func parsePlist(name: String) -> Self?{
let path = NSBundle.mainBundle().pathForResource(name+".plist", ofType: nil)
if path == nil {return nil}
let dict = NSDictionary(contentsOfFile: path!)
if dict == nil {return nil}
return parse(dict: dict!)
}
class func parses(arr arr: NSArray) -> [Reflect]{
var models: [Reflect] = []
for (_ , dict) in arr.enumerate(){
let model = self.parse(dict: dict as! NSDictionary)
models.append(model)
}
return models
}
class func parse(dict dict: NSDictionary) -> Self{
let mutableDict = NSMutableDictionary.init(dictionary:dict as! [NSObject:AnyObject]);
let model = self.init()
let mappingDict = model.mappingDict()
let ignoreProperties = model.ignorePropertiesForParse()
model.properties { (name, type, value) -> Void in
let dataDictHasKey = dict[name] != nil
let mappdictDictHasKey = mappingDict?[name] != nil
let needIgnore = ignoreProperties == nil ? false : (ignoreProperties!).contains(name)
if (dataDictHasKey || mappdictDictHasKey) && !needIgnore {
let key = mappdictDictHasKey ? mappingDict![name]! : name
if !type.isArray {
if !type.isReflect {
if type.typeClass == Bool.self { //bool
model.setValue(dict[key]?.boolValue, forKeyPath: name)
}else{
if type.typeClass == NSNumber.self{
let tempInfo = dict[name];
print("temp info infoiii :\(tempInfo)");
}else{
print("value:\(dict[key])--path:\(name)");
let valueInfo = dict[key];
if(name == "buy_time" || name == "order_id" || name == "email"){
if (name == "buy_time" || name == "email"){
if (valueInfo?.classForCoder == NSNull.classForCoder()){
model.setValue("", forKey: key);
}}
if name == "order_id"{
if (valueInfo?.classForCoder == NSNull.classForCoder()){
model.setValue(NSNumber.init(int: -1), forKey: key);
}
}
}else{
if dict[key] == nil || name == "resume_status"{
model.setValue(NSNumber.init(int: -1), forKey: key);
}else{
model.setValue(dict[key], forKeyPath: name)}
}
}
// print("model typeinfo \(dict)--type:\(type.typeName)--class:\(type.typeClass)")
//
// let testTemp = dict[name];
// print("test temp class :\(testTemp?.classForCoder)---name:\(name)");
//
// print("class info :\(type.typeClass)");
//
//
// print("compare result tyoeclass:\(type.typeClass)--numberclass:\(NSNumber.classForCoder())---realType:\(type.realType)");
//
// if (GNUserService.sharedUserService().swiftComparCateWithString(type.typeName)){
//
// print("dict name :\(dict[name])--name:\(name)");
//
// // let modelInfo = String.init(format: "%@", dict[name] as! NSNumber);
//
// let modelInfo = GNUserService.sharedUserService().swiftTransportInfo(dict[name]);
//
// print("model uiteminfo :\(modelInfo)");
// let numberNull = NSNumber.init(int: -1) as AnyObject;
// if( modelInfo == "<null>"){
// dict.setValue(numberNull, forKeyPath: name);
// }
// }else{
// model.setValue(dict[key], forKeyPath: name)
// }
}
}else{
//这里是模型
//首选判断字典中是否有值
let dictValue = dict[key]
if dictValue != nil { //字典中有模型
let modelValue = model.valueForKeyPath(key)
if modelValue != nil { //子模型已经初始化
model.setValue((type.typeClass as! Reflect.Type).parse(dict: dict[key] as! NSDictionary), forKeyPath: name)
}else{ //子模型没有初始化
//先主动初始化
let cls = ClassFromString(type.typeName)
model.setValue((cls as! Reflect.Type).parse(dict: dict[key] as! NSDictionary), forKeyPath: name)
}
}
}
}else{
if let res = type.isAggregate(){
var arrAggregate = []
if res is Int.Type {
arrAggregate = parseAggregateArray(dict[key] as! NSArray, basicType: ReflectType.BasicType.Int, ins: 0)
}else if res is Float.Type {
arrAggregate = parseAggregateArray(dict[key] as! NSArray, basicType: ReflectType.BasicType.Float, ins: 0.0)
}else if res is Double.Type {
arrAggregate = parseAggregateArray(dict[key] as! NSArray, basicType: ReflectType.BasicType.Double, ins: 0.0)
}else if res is String.Type {
arrAggregate = parseAggregateArray(dict[key] as! NSArray, basicType: ReflectType.BasicType.String, ins: "")
}else if res is NSNumber.Type {
arrAggregate = parseAggregateArray(dict[key] as! NSArray, basicType: ReflectType.BasicType.NSNumber, ins: NSNumber())
}else{
arrAggregate = dict[key] as! [AnyObject]
}
print("arrdsfdj :\(arrAggregate)");
model.setValue(arrAggregate, forKeyPath: name)
}else{
let elementModelType = ReflectType.makeClass(type) as! Reflect.Type
let dictKeyArr = dict[key] as! NSArray
var arrM: [Reflect] = []
for (_, value) in dictKeyArr.enumerate() {
let elementModel = elementModelType.parse(dict: value as! NSDictionary)
arrM.append(elementModel)
}
model.setValue(arrM, forKeyPath: name)
}
}
}
}
model.parseOver()
return model
}
class func parseAggregateArray<T>(arrDict: NSArray,basicType: ReflectType.BasicType, ins: T) -> [T]{
var intArrM: [T] = []
if arrDict.count == 0 {return intArrM}
for (_, value) in arrDict.enumerate() {
var element: T = ins
let v = "\(value)"
if T.self is Int.Type {
element = Int(Float(v)!) as! T
}
else if T.self is Float.Type {element = v.floatValue as! T}
else if T.self is Double.Type {element = v.doubleValue as! T}
else if T.self is NSNumber.Type {element = NSNumber(double: v.doubleValue!) as! T}
else{element = value as! T}
intArrM.append(element)
}
return intArrM
}
func mappingDict() -> [String: String]? {return nil}
func ignorePropertiesForParse() -> [String]? {return nil}
}
| mit | a8dcd2467875c15e476eb21e8b740362 | 36.822314 | 145 | 0.41429 | 5.454708 | false | false | false | false |
pvzig/SlackKit | SKRTMAPI/Sources/Conformers/VaporEngineRTM.swift | 1 | 2776 | //
// VaporEngineRTM.swift
//
// Copyright © 2017 Peter Zignego. All rights reserved.
//
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in
// all copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
// THE SOFTWARE.
#if os(Linux) || os(macOS) && !COCOAPODS
import Foundation
import NIO
import WebSocketKit
// Builds with *Swift Package Manager ONLY*
public class VaporEngineRTM: RTMWebSocket {
private let eventLoopGroup = MultiThreadedEventLoopGroup(numberOfThreads: 1)
// Delegate
public weak var delegate: RTMDelegate?
// Websocket
private var websocket: WebSocket?
private var futureWebsocket: EventLoopFuture<Void>?
public required init() {}
public func connect(url: URL) {
guard let host = url.host else {
fatalError("ERROR - Cannot extract host from '\(url.absoluteString)'")
}
futureWebsocket = WebSocketClient(eventLoopGroupProvider: .shared(eventLoopGroup))
.connect(scheme: url.scheme ?? "ws", host: host, port: 8080, path: url.path, query: nil, headers: [:]) { [weak self] webSocket in
self?.didConnect(websocket: webSocket)
}
}
func didConnect(websocket: WebSocket) {
self.websocket = websocket
delegate?.didConnect()
websocket.onText { ws, text in
self.delegate?.receivedMessage(text)
}
websocket.onClose
.whenComplete { [weak self] _ in
self?.delegate?.disconnected()
}
}
public func disconnect() {
_ = websocket?.close()
websocket = nil
futureWebsocket = nil
}
public func sendMessage(_ message: String) throws {
guard let websocket = websocket else { throw SlackError.rtmConnectionError }
websocket.send(message)
}
}
#endif
| mit | 99c295d88e9a3667909982601b4fd879 | 34.576923 | 141 | 0.684324 | 4.70339 | false | false | false | false |
danimal141/TagListView | TagListView/TagListView.swift | 5 | 6464 | //
// TagListView.swift
// TagListViewDemo
//
// Created by Dongyuan Liu on 2015-05-09.
// Copyright (c) 2015 Ela. All rights reserved.
//
import UIKit
@objc public protocol TagListViewDelegate {
optional func tagPressed(title: String, tagView: TagView, sender: TagListView) -> Void
}
@IBDesignable
public class TagListView: UIView {
@IBInspectable public var textColor: UIColor = UIColor.whiteColor() {
didSet {
for tagView in tagViews {
tagView.textColor = textColor
}
}
}
@IBInspectable public var tagBackgroundColor: UIColor = UIColor.grayColor() {
didSet {
for tagView in tagViews {
tagView.tagBackgroundColor = tagBackgroundColor
}
}
}
@IBInspectable public var tagSelectedBackgroundColor: UIColor = UIColor.redColor() {
didSet {
for tagView in tagViews {
tagView.tagSelectedBackgroundColor = tagSelectedBackgroundColor
}
}
}
@IBInspectable public var cornerRadius: CGFloat = 0 {
didSet {
for tagView in tagViews {
tagView.cornerRadius = cornerRadius
}
}
}
@IBInspectable public var borderWidth: CGFloat = 0 {
didSet {
for tagView in tagViews {
tagView.borderWidth = borderWidth
}
}
}
@IBInspectable public var borderColor: UIColor? {
didSet {
for tagView in tagViews {
tagView.borderColor = borderColor
}
}
}
@IBInspectable public var paddingY: CGFloat = 2 {
didSet {
for tagView in tagViews {
tagView.paddingY = paddingY
}
rearrangeViews()
}
}
@IBInspectable public var paddingX: CGFloat = 5 {
didSet {
for tagView in tagViews {
tagView.paddingX = paddingX
}
rearrangeViews()
}
}
@IBInspectable public var marginY: CGFloat = 2 {
didSet {
rearrangeViews()
}
}
@IBInspectable public var marginX: CGFloat = 5 {
didSet {
rearrangeViews()
}
}
public var textFont: UIFont = UIFont.systemFontOfSize(12) {
didSet {
for tagView in tagViews {
tagView.textFont = textFont
}
rearrangeViews()
}
}
@IBOutlet public var delegate: TagListViewDelegate?
var tagViews: [TagView] = []
var tagViewHeight: CGFloat = 0
var rows = 0 {
didSet {
invalidateIntrinsicContentSize()
}
}
// MARK: - Interface Builder
public override func prepareForInterfaceBuilder() {
addTag("Welcome")
addTag("to")
addTag("TagListView").selected = true
}
// MARK: - Layout
public override func layoutSubviews() {
super.layoutSubviews()
rearrangeViews()
}
private func rearrangeViews() {
for tagView in tagViews {
tagView.removeFromSuperview()
}
var currentRow = 0
var currentRowTagCount = 0
var currentRowWidth: CGFloat = 0
for tagView in tagViews {
tagView.frame.size = tagView.intrinsicContentSize()
tagViewHeight = tagView.frame.height
if currentRowTagCount == 0 || currentRowWidth + tagView.frame.width + marginX > frame.width {
currentRow += 1
tagView.frame.origin.x = 0
tagView.frame.origin.y = CGFloat(currentRow - 1) * (tagViewHeight + marginY)
currentRowTagCount = 1
currentRowWidth = tagView.frame.width + marginX
}
else {
tagView.frame.origin.x = currentRowWidth
tagView.frame.origin.y = CGFloat(currentRow - 1) * (tagViewHeight + marginY)
currentRowTagCount += 1
currentRowWidth += tagView.frame.width + marginX
}
addSubview(tagView)
}
rows = currentRow
}
// MARK: - Manage tags
public override func intrinsicContentSize() -> CGSize {
var height = CGFloat(rows) * (tagViewHeight + marginY)
if rows > 0 {
height -= marginY
}
return CGSizeMake(frame.width, height)
}
public func addTag(title: String) -> TagView {
let tagView = TagView(title: title)
tagView.textColor = textColor
tagView.tagBackgroundColor = tagBackgroundColor
tagView.tagSelectedBackgroundColor = tagSelectedBackgroundColor
tagView.cornerRadius = cornerRadius
tagView.borderWidth = borderWidth
tagView.borderColor = borderColor
tagView.paddingY = paddingY
tagView.paddingX = paddingX
tagView.textFont = textFont
tagView.addTarget(self, action: "tagPressed:", forControlEvents: UIControlEvents.TouchUpInside)
return addTagView(tagView)
}
public func addTagView(tagView: TagView) -> TagView {
tagViews.append(tagView)
rearrangeViews()
return tagView
}
public func removeTag(title: String) {
// loop the array in reversed order to remove items during loop
for index in stride(from: tagViews.count - 1, through: 0, by: -1) {
let tagView = tagViews[index]
if tagView.currentTitle == title {
removeTagView(tagView)
}
}
}
public func removeTagView(tagView: TagView) {
tagView.removeFromSuperview()
if let index = find(tagViews, tagView) {
tagViews.removeAtIndex(index)
}
rearrangeViews()
}
public func removeAllTags() {
for tagView in tagViews {
tagView.removeFromSuperview()
}
tagViews = []
rearrangeViews()
}
public func selectedTags() -> [TagView] {
return tagViews.filter() { $0.selected == true }
}
// MARK: - Events
func tagPressed(sender: TagView!) {
sender.onTap?(sender)
delegate?.tagPressed?(sender.currentTitle ?? "", tagView: sender, sender: self)
}
}
| mit | 75d75d9014f40672abf9cb82723d4c55 | 27.227074 | 105 | 0.554765 | 5.368771 | false | false | false | false |
exyte/Macaw | Example/Example/Examples/Animations/AnimationsView.swift | 1 | 2942 | import UIKit
import Macaw
class AnimationsView: MacawView {
var animation: Animation?
var ballNodes = [Group]()
var onComplete: (() -> ()) = {}
let n = 100
let speed = 20.0
let r = 10.0
let ballColors = [
Color(val: 0x1abc9c),
Color(val: 0x2ecc71),
Color(val: 0x3498db),
Color(val: 0x9b59b6),
Color(val: 0xf1c40f),
Color(val: 0xe67e22),
Color(val: 0xe67e22),
Color(val: 0xe74c3c)
]
required init?(coder aDecoder: NSCoder) {
super.init(node: Group(), coder: aDecoder)
}
func startAnimation() {
if let animation = self.animation {
animation.play()
}
}
func prepareAnimation() {
ballNodes.removeAll()
var animations = [Animation]()
let screenBounds = UIScreen.main.bounds
let startPos = Transform.move(
dx: Double(screenBounds.width / 2) - r,
dy: Double(screenBounds.height / 2) - r
)
var velocities = [Point]()
var positions = [Point]()
func posForTime(_ t: Double, index: Int) -> Point {
let prevPos = positions[index]
var velocity = velocities[index]
var pos = prevPos.add(velocity)
// Borders
if pos.x < Double(self.bounds.width) / -2.0 || pos.x > Double(self.bounds.width) / 2.0 {
velocity = Point(x: -1.0 * velocity.x, y: velocity.y)
velocities[index] = velocity
pos = prevPos.add(velocity)
}
if pos.y < Double(self.bounds.height) / -2.0 || pos.y > Double(self.bounds.height) / 2.0 {
velocity = Point(x: velocity.x, y: -1.0 * velocity.y)
velocities[index] = velocity
pos = prevPos.add(velocity)
}
return pos
}
for i in 0 ... (n - 1) {
// Node
let circle = Circle(cx: r, cy: r, r: r)
let shape = Shape(
form: circle,
fill: ballColors[Int(arc4random() % 7)]
)
let ballGroup = Group(contents: [shape])
ballNodes.append(ballGroup)
// Animation
let velocity = Point(
x: -0.5 * speed + speed * Double(arc4random() % 1000) / 1000.0,
y: -0.5 * speed + speed * Double(arc4random() % 1000) / 1000.0)
velocities.append(velocity)
positions.append(Point(x: 0.0, y: 0.0))
let anim = ballGroup.placeVar.animation({ (t) -> Transform in
let pos = posForTime(t, index: i)
positions[i] = pos
return Transform().move(
dx: pos.x,
dy: pos.y)
}, during: 3.0)
animations.append([
anim,
ballGroup.opacityVar.animation((0.1 >> 1.0).t(3.0))
].combine())
}
animation = animations.combine().autoreversed().onComplete {
self.completeAnimation()
}
let node = Group(contents: ballNodes)
node.place = Transform().move(dx: startPos.dx, dy: startPos.dy)
self.node = node
}
func completeAnimation() {
self.node = Group()
self.prepareAnimation()
self.onComplete()
}
}
| mit | 6e72e58e140ab8d4d317aab2c14a34d1 | 23.722689 | 93 | 0.574099 | 3.194354 | false | false | false | false |
TheInfiniteKind/duckduckgo-iOS | Core/UserText.swift | 1 | 2757 | //
// UserText.swift
// DuckDuckGo
//
// Created by Mia Alexiou on 24/01/2017.
// Copyright © 2017 DuckDuckGo. All rights reserved.
//
import Foundation
public struct UserText {
public static let appTitle = forKey("app.title")
public static let appInfo = forKey("app.info")
public static let appInfoWithBuild = forKey("app.infoWithBuild")
public static let homeLinkTitle = forKey("home.link.title")
public static let searchDuckDuckGo = forKey("search.hint.duckduckgo")
public static let webSessionCleared = forKey("web.session.clear")
public static let webSaveLinkDone = forKey("web.url.save.done")
public static let onboardingRealPrivacyTitle = forKey("onboarding.realprivacy.title")
public static let onboardingRealPrivacyDescription = forKey( "onboarding.realprivacy.description")
public static let onboardingContentBlockingTitle = forKey("onboarding.contentblocking.title")
public static let onboardingContentBlockingDescription = forKey("onboarding.contentblocking.description")
public static let onboardingTrackingTitle = forKey("onboarding.tracking.title")
public static let onboardingTrackingDescription = forKey("onboarding.tracking.description")
public static let onboardingPrivacyRightTitle = forKey("onboarding.privacyright.title")
public static let onboardingPrivacyRightDescription = forKey("onboarding.privacyright.description")
public static let feedbackEmailSubject = forKey("feedbackemail.subject")
public static let feedbackEmailBody = forKey("feedbackemail.body")
public static let actionSave = forKey("action.title.save")
public static let actionCancel = forKey("action.title.cancel")
public static let actionNewTab = forKey("action.title.newTab")
public static let actionOpen = forKey("action.title.open")
public static let actionReadingList = forKey("action.title.readingList")
public static let actionCopy = forKey("action.title.copy")
public static let actionShare = forKey("action.title.share")
public static let actionSaveBookmark = forKey("action.title.save.bookmark")
public static let alertSaveBookmark = forKey("alert.title.save.bookmark")
public static let alertEditBookmark = forKey("alert.title.edit.bookmark")
public static let navigationTitleEdit = forKey("navigation.title.edit")
public static func forDateFilter(_ dateFilter: DateFilter) -> String {
if dateFilter == .any {
return forKey("datefilter.code.any")
}
let key = "datefilter.code.\(dateFilter.rawValue)"
return forKey(key)
}
fileprivate static func forKey(_ key: String) -> String {
return NSLocalizedString(key, comment: key)
}
}
| apache-2.0 | 255b33de41aaff41ea800c824fd29e75 | 44.180328 | 109 | 0.737663 | 4.631933 | false | false | false | false |
moritzsternemann/SwipyCell | Sources/SwipyCell/SwipyCell.swift | 1 | 19323 | //
// SwipyCell.swift
// SwipyCell
//
// Created by Moritz Sternemann on 17.01.16.
// Copyright © 2016 Moritz Sternemann. All rights reserved.
//
import UIKit
fileprivate struct SwipyCellConstants {
static let bounceAmplitude = 20.0 // Maximum bounce amplitude whe using the switch mode
static let damping: CGFloat = 0.6 // Damping of the spring animation
static let velocity: CGFloat = 0.9 // Velocity of the spring animation
static let animationDuration = 0.4 // Duration of the animation
static let bounceDuration1 = 0.2 // Duration of the first part of the bounce animation
static let bounceDuration2 = 0.1 // Duration of the second part of the bounce animation
static let durationLowLimit = 0.25 // Lowest duration when swiping the cell because we try to simulate velocity
static let durationHighLimit = 0.1 // Highest duration when swiping the cell because we try to simulate velocity
}
open class SwipyCell: UITableViewCell, SwipyCellTriggerPointEditable {
public var delegate: SwipyCellDelegate?
public var shouldAnimateSwipeViews: Bool!
public var defaultColor: UIColor!
public var swipeViewPadding: CGFloat!
var panGestureRecognizer: UIPanGestureRecognizer!
var isExited: Bool!
var isDragging: Bool!
var shouldDrag: Bool!
var currentPercentage: CGFloat!
var direction: SwipyCellDirection!
var damping: CGFloat!
var velocity: CGFloat!
var animationDuration: TimeInterval!
var contentScreenshotView: UIImageView?
var colorIndicatorView: UIView!
var slidingView: UIView!
var activeView: UIView?
fileprivate(set) public var triggers: [SwipyCellState: SwipyCellTrigger] = [:] {
didSet { updateTriggerDirections() }
}
public var triggerPoints: [CGFloat: SwipyCellState] = [:] {
didSet { updateFirstTriggerPoints() }
}
var triggerDirections: Set<SwipyCellDirection> = []
var firstLeftTrigger: CGFloat!
var firstRightTrigger: CGFloat!
// MARK: - Initialization
override public init(style: UITableViewCell.CellStyle, reuseIdentifier: String?) {
super.init(style: style, reuseIdentifier: reuseIdentifier)
initializer()
}
required public init?(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)
initializer()
}
func initializer() {
initDefaults()
panGestureRecognizer = UIPanGestureRecognizer(target: self, action: #selector(handlePanGesture))
addGestureRecognizer(panGestureRecognizer)
panGestureRecognizer.delegate = self
}
func initDefaults() {
let cfg = SwipyCellConfig.shared
shouldAnimateSwipeViews = cfg.shouldAnimateSwipeViews
defaultColor = cfg.defaultSwipeViewColor
swipeViewPadding = SwipyCellConfig.shared.swipeViewPadding
isExited = false
isDragging = false
shouldDrag = true
damping = SwipyCellConstants.damping
velocity = SwipyCellConstants.velocity
animationDuration = SwipyCellConstants.animationDuration
activeView = nil
triggerPoints = SwipyCellConfig.shared.triggerPoints
}
func setupSwipeView() {
if contentScreenshotView != nil {
return
}
let contentViewScreenshotImage = image(withView: self)
colorIndicatorView = UIView(frame: bounds)
colorIndicatorView.autoresizingMask = [.flexibleHeight, .flexibleWidth]
addSubview(colorIndicatorView)
slidingView = UIView()
slidingView.contentMode = .center
colorIndicatorView.addSubview(slidingView)
contentScreenshotView = UIImageView(image: contentViewScreenshotImage)
addSubview(contentScreenshotView!)
}
// MARK: - Public Interface
public func addSwipeTrigger(forState state: SwipyCellState, withMode mode: SwipyCellMode, swipeView view: UIView, swipeColor color: UIColor, completion block: SwipyCellTriggerBlock?) {
triggers[state] = SwipyCellTrigger(mode: mode, color: color, view: view, block: block)
}
public func setCompletionBlock(forState state: SwipyCellState, _ block: @escaping SwipyCellTriggerBlock) {
guard triggers[state] != nil else { return }
triggers[state]?.block = block
}
public func setCompletionBlocks(_ block: SwipyCellTriggerBlock?) {
for trigger in triggers {
triggers[trigger.key]?.block = block
}
}
// MARK: - Prepare reuse
override open func prepareForReuse() {
super.prepareForReuse()
uninstallSwipeView()
initDefaults()
}
func uninstallSwipeView() {
if contentScreenshotView == nil {
return
}
slidingView.removeFromSuperview()
slidingView = nil
colorIndicatorView.removeFromSuperview()
colorIndicatorView = nil
contentScreenshotView!.removeFromSuperview()
contentScreenshotView = nil
}
// MARK: - Gesture Recognition
@objc func handlePanGesture(_ gesture: UIPanGestureRecognizer) {
if shouldDrag == false || isExited == true {
return
}
let state = gesture.state
let translation = gesture.translation(in: self)
let velocity = gesture.velocity(in: self)
var percentage: CGFloat = 0.0
if let contentScreenshotView = contentScreenshotView {
percentage = swipePercentage(withOffset: contentScreenshotView.frame.minX, relativeToWidth: bounds.width)
}
let animationDuration = viewAnimationDuration(withVelocity: velocity)
direction = swipeDirection(withPercentage: percentage)
let cellState = swipeState(withPercentage: percentage)
let stateTriggerHit = triggerHit(withPercentage: percentage)
if state == .began || state == .changed {
isDragging = true
setupSwipeView()
let center = CGPoint(x: (contentScreenshotView?.center.x ?? 0) + translation.x, y: contentScreenshotView?.center.y ?? 0)
contentScreenshotView?.center = center
animate(withOffset: contentScreenshotView?.frame.minX ?? 0)
gesture.setTranslation(.zero, in: self)
delegate?.swipyCell(self, didSwipeWithPercentage: percentage, currentState: cellState, triggerActivated: stateTriggerHit)
} else if state == .ended || state == .cancelled {
isDragging = false
activeView = swipeView(withSwipeState: cellState)
currentPercentage = percentage
let cellMode = triggers[cellState]?.mode ?? .none
if stateTriggerHit && cellMode == .exit && direction != .center {
move(withDuration: animationDuration, inDirection: direction)
} else {
swipeToOrigin {
if stateTriggerHit {
self.executeTriggerBlock()
}
}
}
delegate?.swipyCellDidFinishSwiping(self, atState: cellState, triggerActivated: stateTriggerHit)
}
}
open override func gestureRecognizerShouldBegin(_ gestureRecognizer: UIGestureRecognizer) -> Bool {
guard let gesture = gestureRecognizer as? UIPanGestureRecognizer else { return false }
let point = gesture.velocity(in: self)
if abs(point.x) > abs(point.y) {
// if there are no states for direction (point.x > or < 0) return false
if (point.x > 0 && !triggerDirections.contains(.left))
|| (point.x < 0 && !triggerDirections.contains(.right)) {
return false
}
delegate?.swipyCellDidStartSwiping(self)
return true
}
return false
}
// MARK: - Percentage calculations
func swipeOffset(withPercentage percentage: CGFloat, relativeToWidth width: CGFloat) -> CGFloat {
var offset = percentage * width
if offset < -width {
offset = -width
} else if offset > width {
offset = width
}
return offset
}
func swipePercentage(withOffset offset: CGFloat, relativeToWidth width: CGFloat) -> CGFloat {
var percentage = offset / width
if percentage < -1.0 {
percentage = -1.0
} else if offset > width {
percentage = 1.0
}
return percentage
}
// MARK: - Animation calculations
func viewAnimationDuration(withVelocity velocity: CGPoint) -> TimeInterval {
let width = bounds.width
let animationDurationDiff = SwipyCellConstants.durationHighLimit - SwipyCellConstants.durationLowLimit
var horizontalVelocity = velocity.x
if horizontalVelocity < -width {
horizontalVelocity = -width
} else if horizontalVelocity > width {
horizontalVelocity = width
}
return TimeInterval(SwipyCellConstants.durationHighLimit + SwipyCellConstants.durationLowLimit - fabs(Double(horizontalVelocity / width) * animationDurationDiff))
}
// MARK: - State calculations
func swipeDirection(withPercentage percentage: CGFloat) -> SwipyCellDirection {
if percentage < 0 {
return .left
} else if percentage > 0 {
return .right
}
return .center
}
func swipeState(withPercentage percentage: CGFloat) -> SwipyCellState {
if percentage == 0.0 {
return .none
}
// Swipe left (right trigger points): percentage < 0, Swipe right (left trigger points): percentage > 0
// x: = 0
// +: > 0, >= trigger[x]
// -: < 0, <= trigger[x]
let keys = Array(triggerPoints.keys).sorted()
for (i, key) in keys.enumerated() {
if (percentage > 0 && key < 0) || (percentage < 0 && key > 0) {
continue
}
if percentage > 0 {
let nextKey = (keys.count > i + 1) ? keys[i + 1] : 1
let nextStateAvailable = (triggers[triggerPoints[nextKey] ?? .none] != nil)
// positive trigger matches
if (percentage >= key && (!nextStateAvailable || percentage < nextKey)) || (percentage < firstLeftTrigger && key == firstLeftTrigger) {
return triggerPoints[key] ?? .none
}
} else { // percentage < 0
let nextKey = (i - 1 >= 0) ? keys[i - 1] : -1
let nextStateAvailable = (triggers[triggerPoints[nextKey] ?? .none] != nil)
// negative trigger matches
if (percentage <= key && (!nextStateAvailable || percentage > nextKey)) || (percentage > firstRightTrigger && key == firstRightTrigger) {
return triggerPoints[key] ?? .none
}
}
}
return .none
}
func swipeView(withSwipeState state: SwipyCellState) -> UIView? {
return triggers[state]?.view
}
func swipeColor(withSwipeState state: SwipyCellState) -> UIColor {
return triggers[state]?.color ?? defaultColor
}
func swipeAlpha(withPercentage percentage: CGFloat) -> CGFloat {
var alpha: CGFloat = 1.0
if percentage >= 0 && percentage < firstLeftTrigger {
alpha = percentage / firstLeftTrigger
} else if percentage < 0 && percentage > firstRightTrigger {
alpha = abs(percentage / abs(firstRightTrigger))
}
return alpha
}
// MARK: - Trigger handling
func updateTriggerDirections() {
triggerDirections = []
for state in triggers.keys {
switch state {
case .none:
continue
case .state(_, let direction):
triggerDirections.insert(direction)
break
}
}
}
func updateFirstTriggerPoints() {
firstLeftTrigger = firstTrigger(forDirection: .left)
firstRightTrigger = firstTrigger(forDirection: .right)
}
// MARK: - Animations / View movement
func animate(withOffset offset: CGFloat) {
let percentage = swipePercentage(withOffset: offset, relativeToWidth: bounds.width)
let state = swipeState(withPercentage: percentage)
let view = swipeView(withSwipeState: state)
if let view = view {
setView(ofSlidingView: view)
slidingView.alpha = swipeAlpha(withPercentage: percentage)
slideSwipeView(withPercentage: percentage, view: view, isDragging: shouldAnimateSwipeViews)
}
let color = swipeColor(withSwipeState: state)
colorIndicatorView.backgroundColor = color
}
func slideSwipeView(withPercentage percentage: CGFloat, view: UIView?, isDragging: Bool) {
guard let view = view else { return }
var position: CGPoint = .zero
position.y = bounds.height / 2.0
if isDragging {
if percentage > 0 && percentage < firstLeftTrigger {
position.x = swipeOffset(withPercentage: firstLeftTrigger, relativeToWidth: bounds.width) - view.bounds.width - swipeViewPadding
} else if percentage >= firstLeftTrigger {
position.x = swipeOffset(withPercentage: percentage, relativeToWidth: bounds.width) - view.bounds.width - swipeViewPadding
} else if percentage < 0 && percentage > firstRightTrigger {
position.x = bounds.width + swipeOffset(withPercentage: firstRightTrigger, relativeToWidth: bounds.width) + view.bounds.width + swipeViewPadding
} else if percentage <= firstRightTrigger {
position.x = bounds.width + swipeOffset(withPercentage: percentage, relativeToWidth: bounds.width) + view.bounds.width + swipeViewPadding
}
} else {
if direction == .right {
position.x = swipeOffset(withPercentage: firstLeftTrigger, relativeToWidth: bounds.width) - view.bounds.width - swipeViewPadding
} else if direction == .left {
position.x = bounds.width + swipeOffset(withPercentage: firstRightTrigger, relativeToWidth: bounds.width) + view.bounds.width + swipeViewPadding
} else {
return
}
}
// cap slide view inside visible area
if direction == .right {
position.x = max(position.x, view.bounds.width + swipeViewPadding / 2.0)
} else if direction == .left {
position.x = min(position.x, bounds.width - view.bounds.width - swipeViewPadding / 2.0)
}
let activeViewSize = view.bounds.size
var activeViewFrame = CGRect(x: position.x - activeViewSize.width / 2.0,
y: position.y - activeViewSize.height / 2.0,
width: activeViewSize.width,
height: activeViewSize.height)
activeViewFrame = activeViewFrame.integral
slidingView.frame = activeViewFrame
}
func move(withDuration duration: TimeInterval, inDirection direction: SwipyCellDirection) {
isExited = true
var origin: CGFloat = 0.0
if direction == .left {
origin = -bounds.width
} else if direction == .right {
origin = bounds.width
}
let percentage = swipePercentage(withOffset: origin, relativeToWidth: bounds.width)
var frame = contentScreenshotView?.frame ?? .zero
frame.origin.x = origin
let state = swipeState(withPercentage: currentPercentage)
let color = swipeColor(withSwipeState: state)
colorIndicatorView.backgroundColor = color
UIView.animate(withDuration: duration, delay: 0, options: [.curveEaseOut, .allowUserInteraction], animations: {
self.contentScreenshotView?.frame = frame
self.slidingView.alpha = 0
self.slideSwipeView(withPercentage: percentage, view: self.activeView, isDragging: self.shouldAnimateSwipeViews)
}, completion: { _ in
self.executeTriggerBlock()
})
}
public func swipeToOrigin(_ block: @escaping () -> Void) {
UIView.animate(withDuration: animationDuration, delay: 0, usingSpringWithDamping: damping, initialSpringVelocity: velocity, options: [], animations: {
var frame = self.contentScreenshotView?.frame ?? .zero
frame.origin.x = 0
self.contentScreenshotView?.frame = frame
self.colorIndicatorView.backgroundColor = self.defaultColor
self.slidingView.alpha = 0
self.slideSwipeView(withPercentage: 0, view: self.activeView, isDragging: false)
}, completion: { finished in
self.isExited = false
self.uninstallSwipeView()
if finished {
block()
}
})
}
// MARK: - View setup
func setView(ofSlidingView view: UIView) {
let subviews = slidingView.subviews
_ = subviews.map { view in
view.removeFromSuperview()
}
slidingView.addSubview(view)
}
// MARK: - Utilities
func image(withView view: UIView) -> UIImage {
let scale = UIScreen.main.scale
UIGraphicsBeginImageContextWithOptions(view.bounds.size, false, scale)
view.layer.render(in: UIGraphicsGetCurrentContext()!)
let image = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
return image!
}
func executeTriggerBlock() {
let state = swipeState(withPercentage: currentPercentage)
triggers[state]?.executeTriggerBlock(withSwipyCell: self, state: state)
}
func firstTrigger(forDirection direction: SwipyCellDirection) -> CGFloat {
var ret: CGFloat = (direction == .right) ? -1.0 : 1.0
for (point, state) in triggerPoints {
guard case let .state(_, stateDirection) = state else { continue }
if direction == stateDirection {
if (direction == .left && point < ret)
|| (direction == .right && point > ret) {
ret = point
}
}
}
return ret
}
func triggerHit(withPercentage percentage: CGFloat) -> Bool {
if percentage >= firstLeftTrigger || percentage <= firstRightTrigger {
return true
}
return false
}
}
| mit | 9cb2e5754bd69fbbfeb81877dda65bc6 | 35.80381 | 188 | 0.599058 | 5.178772 | false | false | false | false |
Ryukie/Ryubo | Ryubo/Ryubo/Classes/Tools/RYEmotion/RYEmotionPackage.swift | 1 | 1965 | //
// RYEmotionPackage.swift
// Ryubo
//
// Created by 王荣庆 on 16/2/24.
// Copyright © 2016年 Ryukie. All rights reserved.
//
import UIKit
class RYEmotionPackage: NSObject {
/// 表情包路径名,浪小花的数据需要修改
var id: String?
/// 表情包名称
var group_name_cn: String?
/// 表情包数组
lazy var emotions = [RYEmotionModel]()
init(dict: [String: AnyObject],idStr:String) {
super.init()
var index = 0
id = idStr
group_name_cn = dict["group_name_cn"] as? String
if let array = dict["emoticons"] as? [[String: String]] {
//运行测试,发现无法显示图片,原因是 em.png 中只有图片的名称,而没有图片路径
let dir = id
for var d in array {
//拼接路径
if let png = d["png"] {
d["png"] = dir! + "/" + png
}
// 追加删除按钮
if index == 20 {
emotions.append(RYEmotionModel(isRemoved: true))
index = 0
}
index++
emotions.append(RYEmotionModel(dict: d,id: id!))
}
}
addEmptyButton()
}
/// 追加空白按钮
private func addEmptyButton() {
let count = emotions.count % 21
print("数组剩余按钮 \(count)")
//一页满21 个就什么都不做
if emotions.count > 0 && count == 0 {
return
}
//有count个 从count开始全部空格
for _ in count..<20 {
emotions.append(RYEmotionModel(isEmpty: true))
}
// 最末尾追加删除按钮
emotions.append(RYEmotionModel(isRemoved: true))
}
override var description: String {
let keys = ["id", "group_name_cn", "emotions"]
return dictionaryWithValuesForKeys(keys).description
}
}
| mit | d7bb293b52fe4346fa36050017aa406f | 26.555556 | 68 | 0.50288 | 3.86637 | false | false | false | false |
Intercambio/CloudUI | CloudUI/CloudUI/CloudServiceSettingsInteractor.swift | 1 | 1961 | //
// CloudServiceSettingsInteractor.swift
// CloudUI
//
// Created by Tobias Kraentzer on 04.03.17.
// Copyright © 2017 Tobias Kräntzer. All rights reserved.
//
import Foundation
import CloudService
extension CloudService: SettingsInteractor {
public func values(forAccountWith identifier: AccountID) -> [SettingsKey: Any]? {
do {
guard
let account = try self.account(with: identifier)
else { return nil }
var result: [String: Any] = [:]
result[SettingsKey.Label] = account.label
result[SettingsKey.BaseURL] = account.url
result[SettingsKey.Username] = account.username
return result
} catch {
return nil
}
}
public func password(forAccountWith identifier: AccountID) -> String? {
do {
guard
let account = try account(with: identifier)
else { return nil }
return password(for: account)
} catch {
return nil
}
}
public func update(accountWith identifier: AccountID, using values: [SettingsKey: Any]) throws -> [SettingsKey: Any]? {
guard
let account = try self.account(with: identifier)
else { return values }
let label = values[SettingsKey.Label] as? String
if account.label != label {
try update(account, with: label)
}
return self.values(forAccountWith: identifier)
}
public func setPassword(_ password: String?, forAccountWith identifier: AccountID) throws {
guard
let account = try account(with: identifier)
else { return }
setPassword(password, for: account)
}
public func remove(accountWith identifier: AccountID) throws {
guard
let account = try account(with: identifier)
else { return }
try remove(account)
}
}
| gpl-3.0 | 35642ccfd5506be718b6ab06235c4397 | 29.609375 | 123 | 0.589076 | 4.686603 | false | false | false | false |
Pyroh/Fluor | Fluor/Controllers/ViewControllers/UserNotificationEnablementViewController.swift | 1 | 4008 | //
// UserNotificationEnablementViewController.swift
//
// Fluor
//
// MIT License
//
// Copyright (c) 2020 Pierre Tacchi
//
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all
// copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
// SOFTWARE.
//
import Cocoa
import UserNotifications
final class UserNotificationEnablementViewController: NSViewController, StoryboardInstantiable {
static var storyboardName: NSStoryboard.Name { .preferences }
static var sceneIdentifier: NSStoryboard.SceneIdentifier? { "NotificationsEnablement" }
@IBOutlet weak var trailiingConstraint: NSLayoutConstraint!
@IBOutlet weak var topConstraint: NSLayoutConstraint!
@IBOutlet weak var bottomConstraint: NSLayoutConstraint!
@IBOutlet weak var leadingConstraint: NSLayoutConstraint!
private var stackViewConstraints: [NSLayoutConstraint] {
[trailiingConstraint, topConstraint, bottomConstraint, leadingConstraint]
}
@objc dynamic var activeAppSwitch: Bool = true
@objc dynamic var activeAppFnKey: Bool = true
@objc dynamic var globalFnKey: Bool = true
@objc dynamic var everytime: Bool = true
@objc dynamic var isShownInAlert: Bool = false
@objc dynamic var canEnableNotifications: Bool {
everytime || activeAppSwitch || activeAppFnKey || globalFnKey
}
override func viewWillAppear() {
self.adaptStackConstraints()
guard !isShownInAlert, AppManager.default.userNotificationEnablement != .none else {
if !isShownInAlert { UserNotificationEnablement.none.apply(to: self) }
return
}
UserNotificationHelper.ifAuthorized(perform: {
AppManager.default.userNotificationEnablement.apply(to: self)
}) {
UserNotificationEnablement.none.apply(to: self)
}
}
@IBAction func checkBoxDidChange(_ sender: NSButton?) {
if let tag = sender?.tag, tag == 1 {
activeAppSwitch = everytime
activeAppFnKey = everytime
globalFnKey = everytime
} else {
everytime = activeAppSwitch && activeAppFnKey && globalFnKey
}
guard !isShownInAlert else { return }
self.checkAuthorizations()
}
private func adaptStackConstraints() {
stackViewConstraints.forEach { $0.constant = isShownInAlert ? 2 : 0 }
}
private func checkAuthorizations() {
UserNotificationHelper.askUser { (isAuthorized) in
if isAuthorized {
AppManager.default.userNotificationEnablement = .from(self)
} else {
UserNotificationEnablement.none.apply(to: self)
}
}
}
override class func keyPathsForValuesAffectingValue(forKey key: String) -> Set<String> {
guard key == "canEnableNotifications" else {
return super.keyPathsForValuesAffectingValue(forKey: key)
}
return .init(["activeAppSwitch", "activeAppFnKey", "globalFnKey", "everytime"])
}
}
| mit | 5c6fcc6dfe2a7e1842ffba01339a7c63 | 38.294118 | 96 | 0.691118 | 4.899756 | false | false | false | false |
cocoascientist/Passengr | Passengr/HTML.swift | 1 | 3065 | //
// HTML.swift
// Passengr
//
// Created by Andrew Shepard on 11/17/15.
// Copyright © 2015 Andrew Shepard. All rights reserved.
//
import Foundation
final class HTMLNode {
internal let nodePtr: xmlNodePtr
private let node: xmlNode
init(node nodePtr: xmlNodePtr) {
self.nodePtr = nodePtr
self.node = nodePtr.pointee
}
lazy var nodeValue: String = {
let document = self.nodePtr.pointee.doc
let children = self.nodePtr.pointee.children
guard let textValue = xmlNodeListGetString(document, children, 1) else { return "" }
defer { free(textValue) }
let value = textValue.withMemoryRebound(to: CChar.self, capacity: 1) {
return String(validatingUTF8: $0)
}
guard value != nil else { return "" }
return value!
}()
}
extension HTMLNode {
func evaluate(xpath: String) -> [HTMLNode] {
let document = nodePtr.pointee.doc
guard let context = xmlXPathNewContext(document) else { return [] }
defer { xmlXPathFreeContext(context) }
context.pointee.node = nodePtr
guard let result = xmlXPathEvalExpression(xpath, context) else { return [] }
defer { xmlXPathFreeObject(result) }
guard let nodeSet = result.pointee.nodesetval else { return [] }
if nodeSet.pointee.nodeNr == 0 || nodeSet.pointee.nodeTab == nil {
return []
}
let size = Int(nodeSet.pointee.nodeNr)
let nodes: [HTMLNode] = [Int](0..<size).compactMap {
guard let node = nodeSet.pointee.nodeTab[$0] else { return nil }
return HTMLNode(node: node)
}
return nodes
}
}
final class HTMLDoc {
final let documentPtr: htmlDocPtr
init?(data: Data) {
let cfEncoding = CFStringConvertNSStringEncodingToEncoding(String.Encoding.utf8.rawValue)
let cfEncodingAsString = CFStringConvertEncodingToIANACharSetName(cfEncoding)
let cEncoding = CFStringGetCStringPtr(cfEncodingAsString, 0)
// HTML_PARSE_RECOVER | HTML_PARSE_NOERROR | HTML_PARSE_NOWARNING
let htmlParseOptions: CInt = 1 << 0 | 1 << 5 | 1 << 6
var ptr: htmlDocPtr? = nil
data.withUnsafeBytes { result -> Void in
guard let bytes = result.baseAddress?.assumingMemoryBound(to: Int8.self) else { return }
ptr = htmlReadMemory(bytes, CInt(data.count), nil, cEncoding, htmlParseOptions)
}
guard let _ = ptr else { return nil }
self.documentPtr = ptr!
}
deinit {
xmlFreeDoc(documentPtr)
self._root = nil
}
private weak var _root: HTMLNode?
var root: HTMLNode? {
if let root = _root {
return root
} else {
guard let root = xmlDocGetRootElement(documentPtr) else { return nil }
let node = HTMLNode(node: root)
self._root = node
return node
}
}
}
| mit | 26106caeb3317a9bb975e9e65de01964 | 29.949495 | 100 | 0.591384 | 4.395983 | false | false | false | false |
radubozga/Freedom | speech/Swift/Speech-gRPC-Streaming/Pods/NVActivityIndicatorView/NVActivityIndicatorView/NVActivityIndicatorView/Animations/NVActivityIndicatorAnimationLineScalePulseOut.swift | 12 | 2737 | //
// NVActivityIndicatorAnimationLineScalePulseOut.swift
// NVActivityIndicatorView
//
// The MIT License (MIT)
// Copyright (c) 2016 Vinh Nguyen
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
// The above copyright notice and this permission notice shall be included in all
// copies or substantial portions of the Software.
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
// SOFTWARE.
//
import UIKit
class NVActivityIndicatorAnimationLineScalePulseOut: NVActivityIndicatorAnimationDelegate {
func setUpAnimation(in layer: CALayer, size: CGSize, color: UIColor) {
let lineSize = size.width / 9
let x = (layer.bounds.size.width - size.width) / 2
let y = (layer.bounds.size.height - size.height) / 2
let duration: CFTimeInterval = 1
let beginTime = CACurrentMediaTime()
let beginTimes = [0.4, 0.2, 0, 0.2, 0.4]
let timingFunction = CAMediaTimingFunction(controlPoints: 0.85, 0.25, 0.37, 0.85)
// Animation
let animation = CAKeyframeAnimation(keyPath: "transform.scale.y")
animation.keyTimes = [0, 0.5, 1]
animation.timingFunctions = [timingFunction, timingFunction]
animation.values = [1, 0.4, 1]
animation.duration = duration
animation.repeatCount = HUGE
animation.isRemovedOnCompletion = false
// Draw lines
for i in 0 ..< 5 {
let line = NVActivityIndicatorShape.line.layerWith(size: CGSize(width: lineSize, height: size.height), color: color)
let frame = CGRect(x: x + lineSize * 2 * CGFloat(i),
y: y,
width: lineSize,
height: size.height)
animation.beginTime = beginTime + beginTimes[i]
line.frame = frame
line.add(animation, forKey: "animation")
layer.addSublayer(line)
}
}
}
| apache-2.0 | 7eada66e302846747a36d7edf5018943 | 41.107692 | 128 | 0.671173 | 4.631134 | false | false | false | false |
IAskWind/IAWExtensionTool | IAWExtensionTool/IAWExtensionTool/Classes/Effect/IAW_FullScreenTool.swift | 1 | 1012 | //
// IAWWeatherTool.swift
// CtkApp
//
// Created by winston on 16/12/14.
// Copyright © 2016年 winston. All rights reserved.
//
import UIKit
open class IAW_FullScreenTool{
/**
*设置全屏显示的效果,在app上加个全局的可穿透的蒙层
* 下雨 下雪 下妹子等
*
*/
open class func showEffect(imgEffect:UIImage,window:UIWindow?){
let snow = ParticleView(imgEffect: imgEffect,frame: CGRect(x: 0, y: 0, width:UIScreen.iawWidth, height: UIScreen.iawHeight))
// snow.imgName = "snowflake1"
// snow.backgroundColor = UIColor.blue
let snowClipView = UIView(frame: snow.frame.offsetBy(dx: 0, dy: 0))
snowClipView.clipsToBounds = true
// snowClipView.backgroundColor = UIColor.red
snowClipView.addSubview(snow)
snow.isUserInteractionEnabled = false //事件穿透
snowClipView.isUserInteractionEnabled = false//事件穿透
window?.addSubview(snowClipView)
}
}
| mit | 68fe9adf76f11262d81efef3073af312 | 29.032258 | 132 | 0.653061 | 3.526515 | false | false | false | false |
PerfectServers/Perfect-Authentication-Server | Sources/PerfectAuthServer/handlers/applications/ApplicationMod.swift | 1 | 1762 | //
// ApplicationMod.swift
// PerfectAuthServer
//
// Created by Jonathan Guthrie on 2017-08-04.
//
import PerfectHTTP
import PerfectLogger
import PerfectLocalAuthentication
extension Handlers {
static func applicationMod(data: [String:Any]) throws -> RequestHandler {
return {
request, response in
let contextAccountID = request.session?.userid ?? ""
let contextAuthenticated = !(request.session?.userid ?? "").isEmpty
if !contextAuthenticated { response.redirect(path: "/login") }
// Verify Admin
Account.adminBounce(request, response)
let obj = Application()
var action = "Create"
if let id = request.urlVariables["id"] {
try? obj.get(id)
if obj.id.isEmpty {
redirectRequest(request, response, msg: "Invalid Application", template: request.documentRoot + "/views/application.mustache")
}
action = "Edit"
}
//show message: it's not saved so all good!
if obj.clientid.isEmpty {
obj.clientid = "To be assigned"
}
if obj.clientsecret.isEmpty {
obj.clientsecret = "To be assigned"
}
var context: [String : Any] = [
"accountID": contextAccountID,
"authenticated": contextAuthenticated,
"mod?":"true",
"action": action,
"name": obj.name,
"clientid": obj.clientid,
"clientsecret": obj.clientsecret,
"id": obj.id
]
for (index, value) in obj.redirecturls.enumerated() {
context["redirect\(index)"] = value
}
if contextAuthenticated {
for i in Handlers.extras(request) {
context[i.0] = i.1
}
}
// add app config vars
for i in Handlers.appExtras(request) {
context[i.0] = i.1
}
response.renderMustache(template: request.documentRoot + "/views/applications.mustache", context: context)
}
}
}
| apache-2.0 | 1403cbbf75deeff16f6b895d323a025a | 22.810811 | 131 | 0.662316 | 3.454902 | false | false | false | false |
jedlewison/AsyncOpKit | Tests/AsyncOpTests/AsyncOpSubclass.swift | 1 | 1469 | //
// AsyncOpInferringInputTests.swift
// AsyncOp
//
// Created by Jed Lewison on 9/3/15.
// Copyright © 2015 Magic App Factory. All rights reserved.
//
import Foundation
import Quick
import Nimble
@testable import AsyncOpKit
let StringForTest = "Subclass Done!"
class AnAsyncOpSubclass : AsyncOp<AsyncVoid, String> {
let testValue: String
required init(testData: String) {
testValue = testData
super.init()
onStart( performOperation )
}
func performOperation(asyncOp: AsyncOp<AsyncVoid, String>) throws -> Void {
usleep(50050)
finish(with: .Some(testValue))
}
}
class AsyncOpSubclassTests : QuickSpec {
override func spec() {
describe("AsyncOp subclass") {
var subject: AnAsyncOpSubclass!
var opQ: NSOperationQueue!
var outputValue: String?
beforeEach {
opQ = NSOperationQueue()
opQ.maxConcurrentOperationCount = 1
subject = AnAsyncOpSubclass(testData: StringForTest)
subject.whenFinished { operation in
outputValue = operation.output.value
}
opQ.addOperation(subject)
}
context("Normal operation") {
it("should out the correct value") {
expect(outputValue).toEventually(equal(StringForTest))
}
}
}
}
}
| mit | b70dade94b18a98b9da7378df6b36282 | 23.881356 | 79 | 0.581744 | 4.926174 | false | true | false | false |
Legoless/Saystack | Code/Extensions/Core/Number+Utilities.swift | 1 | 2405 | //
// Number+Utilities.swift
// Saystack
//
// Created by Dal Rupnik on 30/06/16.
// Copyright © 2016 Unified Sense. All rights reserved.
//
import Foundation
//
// Parts of code taken from post at:
// http://stackoverflow.com/questions/24007129/how-does-one-generate-a-random-number-in-apples-swift-language
//
private func arc4random <T: ExpressibleByIntegerLiteral> (type: T.Type) -> T {
var r: T = 0
arc4random_buf(&r, MemoryLayout<T>.size)
return r
}
public extension UInt64 {
static func random(from: UInt64 = min, to: UInt64 = max) -> UInt64 {
var m: UInt64
let u = to - from
var r = arc4random(type: UInt64.self)
if u > UInt64(Int64.max) {
m = 1 + ~u
} else {
m = ((max - (u * 2)) + 1) % u
}
while r < m {
r = arc4random(type: UInt64.self)
}
return (r % u) + from
}
}
public extension Int64 {
static func random(from: Int64 = min, to: Int64 = max) -> Int64 {
//let (s, overflow) = Int64.subtractWithOverflow(to, from)
let (s, overflow) = to.subtractingReportingOverflow(from)
let u = overflow ? UInt64.max - UInt64(~s) : UInt64(s)
let r = UInt64.random(to: u)
if r > UInt64(Int64.max) {
return Int64(r - (UInt64(~from) + 1))
} else {
return Int64(r) + from
}
}
}
public extension UInt32 {
static func random(from: UInt32 = min, to: UInt32 = max) -> UInt32 {
return arc4random_uniform(to - from) + from
}
}
public extension Int32 {
static func random(from: Int32 = min, to: Int32 = max) -> Int32 {
let r = arc4random_uniform(UInt32(Int64(to) - Int64(from)))
return Int32(Int64(r) + Int64(from))
}
}
public extension UInt {
static func random(from: UInt = min, to: UInt = max) -> UInt {
return UInt(UInt64.random(from: UInt64(from), to: UInt64(to)))
}
}
public extension Int {
static func random(from: Int = min, to: Int = max) -> Int {
return Int(Int64.random(from: Int64(from), to: Int64(to)))
}
}
public extension Float {
static func random() -> Float {
return Float(UInt64.random()) / Float(UInt64.max)
}
}
public extension Double {
static func random() -> Double {
return Double(UInt64.random()) / Double(UInt64.max)
}
}
| mit | 4c85aea6d7cf370d28798e0637bccc1d | 25.130435 | 109 | 0.574459 | 3.297668 | false | false | false | false |
Legoless/Saystack | Code/Extensions/Core/Range+Utilities.swift | 1 | 1229 | //
// Range+Utilities.swift
// Saystack
//
// Created by Dal Rupnik on 23/11/2016.
// Copyright © 2016 Unified Sense. All rights reserved.
//
import Foundation
//
// Original author Martin R. from http://stackoverflow.com/questions/25138339/nsrange-to-rangestring-index
//
public extension String {
func nsRange(from range: Range<String.Index>) -> NSRange {
return NSRange(range, in: self)
/*guard let from = range.lowerBound.samePosition(in: utf16), let to = range.upperBound.samePosition(in: utf16) else {
return NSRange(location: NSNotFound, length: 0)
}
return NSRange(location: utf16.distance(from: utf16.startIndex, to: from), length: utf16.distance(from: from, to: to))*/
}
func range(from nsRange: NSRange) -> Range<String.Index>? {
return Range(nsRange, in: self)
/*guard let from16 = utf16.index(utf16.startIndex, offsetBy: nsRange.location, limitedBy: utf16.endIndex), let to16 = utf16.index(from16, offsetBy: nsRange.length, limitedBy: utf16.endIndex), let from = String.Index(from16, within: self), let to = String.Index(to16, within: self) else { return nil }
return from ..< to*/
}
}
| mit | ce38281ad4af03eb26dbdbc11835c31f | 35.117647 | 308 | 0.660423 | 3.755352 | false | false | false | false |
alloyapple/GooseGtk | Sources/GooseGtk/GTKWidget.swift | 1 | 10987 | //
// Created by color on 17-10-21.
//
import Foundation
import CGTK
import CGooseGtk
import Goose
public enum GTKViewLayer {
case GTKViewLayerBackground
case GTKViewLayerDefault
case GTKViewLayerForeground
case GTKViewLayerNotification
}
public typealias GTKViewDrawingBlock = (UnsafeRawPointer) -> Void
let _GTKKIT_OWNING_VIEW_ = "_GTKKIT_OWNING_VIEW_"
func gtk_widget_get_owning_view(_ widget: UnsafeMutablePointer<GtkWidget>) -> GTKWidget? {
guard let view = g_object_get_data(
CG_OBJECT(widget),
_GTKKIT_OWNING_VIEW_) else {
return nil
}
return Unmanaged<GTKWidget>.fromOpaque(view).takeUnretainedValue()
}
//get-child-position message
let get_child_position = "get-child-position"
typealias get_child_position_func_type = @convention(c) (UnsafeMutablePointer<GtkOverlay>, UnsafeMutablePointer<GtkWidget>, UnsafeMutablePointer<GdkRectangle>, UnsafeRawPointer) -> CInt
//let get_child_position_func: get_child_position_func_type = { (overlay, widget, allocation, user_data) in
// guard let subview = gtk_widget_get_owning_view(widget) else {
// return 0
// }
//
// let view = Unmanaged<GTKView>.fromOpaque(user_data).takeUnretainedValue()
//
// var min = GtkRequisition()
// var req = GtkRequisition()
//
// gtk_widget_get_preferred_size(widget, &min, &req);
//// let frame = view.layoutSubview(subview)
////
////
//// allocation.pointee.x = frame.x;
//// allocation.pointee.y = frame.y;
//// allocation.pointee.width = max(frame.width, min.width);
//// allocation.pointee.height = max(frame.height, min.height);
//
// return 1;
//}
let overlay_widget_destroyed_handler: @convention(c) (UnsafeMutablePointer<GtkWidget>, UnsafeRawPointer) -> Void = { (overlay, view) in
return
}
//let map_handler: @convention(c) (UnsafeMutablePointer<GtkWidget>, UnsafeRawPointer) -> Void = { (overlay, user_data) in
// print("\(#function)")
// let view = Unmanaged<GTKView>.fromOpaque(user_data).takeUnretainedValue()
//
// view.willBecomeMapped()
//
//}
//let map_event_handler: @convention(c) (UnsafeMutablePointer<GtkWidget>, UnsafeRawPointer) -> Void = { (overlay, user_data) in
// print("\(#function)")
// let view = Unmanaged<GTKView>.fromOpaque(user_data).takeUnretainedValue()
//
// view.didBecomeMapped()
//
//}
//let unmap_handler: @convention(c) (UnsafeMutablePointer<GtkWidget>, UnsafeRawPointer) -> Void = { (overlay, user_data) in
// print("\(#function)")
// let view = Unmanaged<GTKView>.fromOpaque(user_data).takeUnretainedValue()
//
// view.willBecomeUnmapped()
//
//}
//let unmap_event_handler: @convention(c) (UnsafeMutablePointer<GtkWidget>, UnsafeRawPointer) -> Void = { (overlay, user_data) in
// print("\(#function)")
// let view = Unmanaged<GTKView>.fromOpaque(user_data).takeUnretainedValue()
//
// view.didBecomeUnmapped()
//
//}
let gesture_drag_begin_handler: @convention(c) (OpaquePointer, Double, Double, UnsafeRawPointer) -> Void = { (gesture, start_x, start_y, user_data) in
}
let gesture_drag_update_handler: @convention(c) (OpaquePointer, Double, Double, UnsafeRawPointer) -> Void = { (gesture, offset_x, offset_y, user_data) in
// guard let view = gtk_widget_get_owning_view(widget) else {
// return 0
// }
//
// let event = GTKEvent()
// event.type = .GTKEventTypeMouseDragged;
//
// var x = 0.0
// var y = 0.0
//
// gtk_gesture_drag_get_start_point(gesture, &x, &y)
// event.originX = UInt32(x)
// event.originY = UInt32(y)
// gtk_gesture_drag_get_offset(gesture, &x, &y)
//
// event.deltaX = x
// event.deltaY = y
//
// event.mouseX = event.originX + UInt32(event.deltaX)
// event.mouseY = event.originY + UInt32(event.deltaY)
//
// view.mouseDragged(event)
}
let gesture_drag_end_handler: @convention(c) (OpaquePointer, Double, Double, UnsafeRawPointer) -> Void = { (gesture, start_x, start_y, user_data) in
}
//let draw_handler: @convention(c) (UnsafeMutablePointer<GtkWidget>, UnsafeRawPointer, UnsafeRawPointer) -> gboolean = { (widget, cr, user_data) in
// guard let view = gtk_widget_get_owning_view(widget) else {
// return 0
// }
//
// view.cairoContext = cr
// view.draw()
//
// view.drawingBlock?(cr)
//
// return 0
//}
//end
public typealias GTKRect = CGTK.GdkRectangle
public typealias Action<Type, Input> = (Type) -> (Input) -> Void
//public class GTKView: GTKResponder {
//
// public var cairoContext: UnsafeRawPointer? = nil
// public var drawingBlock: GTKViewDrawingBlock? = nil
//
// private var observations = [(GTKView) -> Void]()
//
// lazy var mainWidget: UnsafeMutablePointer<GtkWidget> = self.createMainWidget()
// weak var superview: GTKView? = nil
// var hidden: Bool = false
//
// var subViews: [GTKView] = []
//
// override public init() {
// super.init()
//
// g_object_ref_sink(mainWidget)
// gtk_widget_show(mainWidget)
//
// }
//
// var alpha: Double {
// get {
// let alphaValue = gtk_widget_get_opacity(self.mainWidget)
//
// return alphaValue
// }
//
// set {
// gtk_widget_set_opacity(self.mainWidget, newValue)
// }
// }
//
// var tooltip: String {
// get {
// guard let tooltipText = gtk_widget_get_tooltip_text(self.mainWidget) else {
// return ""
// }
//
// return String(cString: tooltipText)
// }
//
// set {
// gtk_widget_set_tooltip_text(self.mainWidget, newValue)
// }
// }
//
// public var name: String {
// get {
// guard let nameString = gtk_widget_get_name(self.mainWidget) else {
// return ""
// }
//
// return String(cString: nameString)
// }
//
// set {
// gtk_widget_set_name(self.mainWidget, newValue)
// }
// }
//
// var frame: GTKRect = GTKRect(x: 0, y: 0, width: 0, height: 0)
//
// public func addTarget<T: AnyObject>(_ target: T,
// action: @escaping Action<T, GTKView>) {
// // We take care of the weak/strong dance for the target, making the API
// // much easier to use and removes the danger of causing retain cycles
// observations.append { [weak target] view in
// guard let target = target else {
// return
// }
//
// // Generate an instance method using the action closure and call it
// action(target)(view)
// }
// }
//
//
// public func draw() {
//
// }
//
// public func removeWidget() {
// gtk_widget_unparent(self.mainWidget)
// }
//
// public func createMainWidget() -> UnsafeMutablePointer<GtkWidget> {
// return gtk_overlay_new()
// }
//
// public func reconnectSignals() {
//
// }
//
// public var hexpand: Bool {
// get {
// return gtk_widget_get_hexpand_set(self.mainWidget) == 1
// }
//
// set {
//
// if newValue {
// gtk_widget_set_hexpand_set(self.mainWidget, 1)
// } else {
// gtk_widget_set_hexpand_set(self.mainWidget, 0)
// }
//
//
// }
// }
//
// public var halign: GtkAlign {
// get {
// return gtk_widget_get_halign(self.mainWidget)
// }
//
// set {
//
// gtk_widget_set_halign(self.mainWidget, newValue)
//
//
// }
// }
//
// public var vexpand: Bool {
// set {
// if newValue {
// gtk_widget_set_vexpand(mainWidget, 1)
// } else {
// gtk_widget_set_vexpand(mainWidget, 0)
// }
// }
//
// get {
// return gtk_widget_get_vexpand(mainWidget) == 1
// }
// }
//
//
// public var sensitive: Bool {
// set {
// if newValue {
// gtk_widget_set_sensitive(mainWidget, 1)
// } else {
// gtk_widget_set_sensitive(mainWidget, 0)
// }
// }
//
// get {
// return gtk_widget_get_sensitive(mainWidget) == 1
// }
// }
//
// public var styleContext: GTKStyleContext {
// get {
// return GTKStyleContext(styleContext: gtk_widget_get_style_context(mainWidget))
// }
// }
//
// public func removeFromSuperView() {
// if let superView = self.superview {
// gtk_container_add(CGTK_CONTAINER(superView.mainWidget), self.mainWidget)
// }
//
// removeFromParent()
// }
//
// public func addChild(_ subView: GTKView) {
// subView.removeFromSuperView()
// subView.superview = self
// self.subViews.append(subView)
// }
//
// public func removeFromParent() {
// if let superView = self.superview {
// superView.subViews.remove(object: self)
// }
// self.superview = nil
// }
//
//
// public func addSubView(_ subView: GTKView) {
// self.addChild(subView)
// gtk_container_add(CGTK_CONTAINER(self.mainWidget), subView.mainWidget)
// }
//
// deinit {
// g_object_unref(self.mainWidget)
// }
//
//}
public class GTKWidget: GTKResponder {
lazy var mainWidget: UnsafeMutablePointer<GtkWidget> = self.createMainWidget()
public var childs: [GTKWidget] = []
override public init() {
super.init()
g_object_ref_sink(self.mainWidget)
self.setOwnView()
}
private func setOwnView() {
g_object_set_data(
self.gobject,
_GTKKIT_OWNING_VIEW_,
Unmanaged.passUnretained(self).toOpaque())
}
public func createMainWidget() -> UnsafeMutablePointer<GtkWidget> {
return gtk_widget_new1(gtk_window_get_type())
}
public func showAll() {
gtk_widget_show_all(self.mainWidget)
}
public func addChild(_ widget: GTKWidget) {
self.childs.append(widget)
}
public var tooltip: String {
get {
guard let tooltipText = gtk_widget_get_tooltip_text(self.mainWidget) else {
return ""
}
return String(cString: tooltipText)
}
set {
gtk_widget_set_tooltip_text(self.mainWidget, newValue)
}
}
public var gobject: UnsafeMutablePointer<GObject> {
return CG_OBJECT(self.mainWidget)
}
public var opaquePointer: gpointer {
return Unmanaged.passUnretained(self).toOpaque()
}
public var name: String {
get {
guard let nameString = gtk_widget_get_name(self.mainWidget) else {
return ""
}
return String(cString: nameString)
}
set {
gtk_widget_set_name(self.mainWidget, newValue)
}
}
deinit {
self.childs.removeAll()
g_object_unref(self.mainWidget)
}
}
| apache-2.0 | 5910bbc1d9b21a2927b13542d2ea243a | 25.667476 | 185 | 0.582416 | 3.513591 | false | false | false | false |
PomTTcat/YYModelGuideRead_JEFF | RxSwiftGuideRead/RxSwift-master/Rx.playground/Pages/Mathematical_and_Aggregate_Operators.xcplaygroundpage/Contents.swift | 5 | 2654 | /*:
> # IMPORTANT: To use **Rx.playground**:
1. Open **Rx.xcworkspace**.
1. Build the **RxExample-macOS** scheme (**Product** → **Build**).
1. Open **Rx** playground in the **Project navigator** (under RxExample project).
1. Show the Debug Area (**View** → **Debug Area** → **Show Debug Area**).
----
[Previous](@previous) - [Table of Contents](Table_of_Contents)
*/
import RxSwift
/*:
# Mathematical and Aggregate Operators
Operators that operate on the entire sequence of items emitted by an `Observable`.
## `toArray`
Converts an `Observable` sequence into an array, emits that array as a new single-element `Observable` sequence, and then terminates. [More info](http://reactivex.io/documentation/operators/to.html)
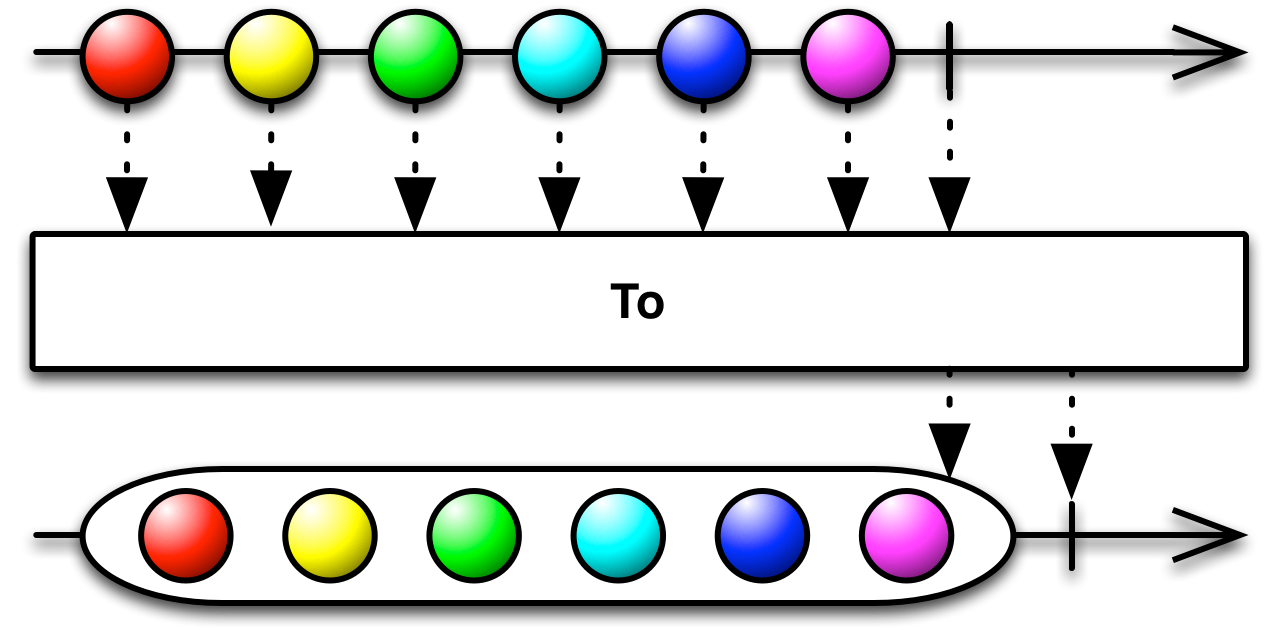
*/
example("toArray") {
let disposeBag = DisposeBag()
Observable.range(start: 1, count: 10)
.toArray()
.subscribe { print($0) }
.disposed(by: disposeBag)
}
/*:
----
## `reduce`
Begins with an initial seed value, and then applies an accumulator closure to all elements emitted by an `Observable` sequence, and returns the aggregate result as a single-element `Observable` sequence. [More info](http://reactivex.io/documentation/operators/reduce.html)
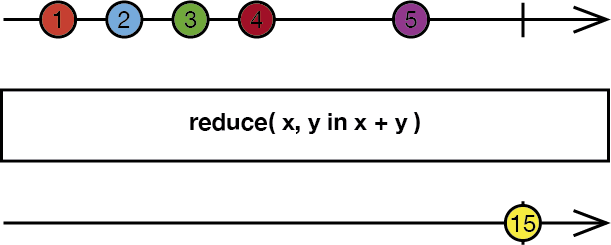
*/
example("reduce") {
let disposeBag = DisposeBag()
Observable.of(10, 100, 1000)
.reduce(1, accumulator: +)
.subscribe(onNext: { print($0) })
.disposed(by: disposeBag)
}
/*:
----
## `concat`
Joins elements from inner `Observable` sequences of an `Observable` sequence in a sequential manner, waiting for each sequence to terminate successfully before emitting elements from the next sequence. [More info](http://reactivex.io/documentation/operators/concat.html)
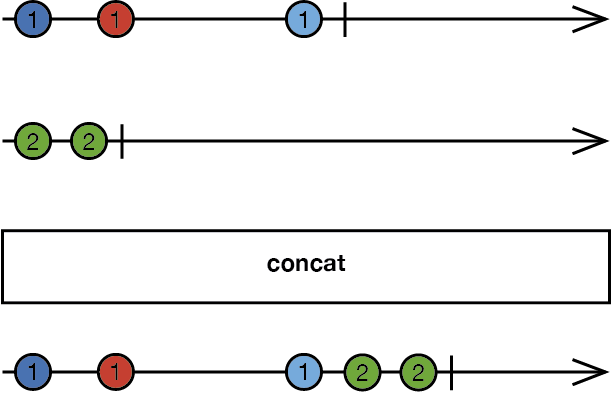
*/
example("concat") {
let disposeBag = DisposeBag()
let subject1 = BehaviorSubject(value: "🍎")
let subject2 = BehaviorSubject(value: "🐶")
let subjectsSubject = BehaviorSubject(value: subject1)
subjectsSubject.asObservable()
.concat()
.subscribe { print($0) }
.disposed(by: disposeBag)
subject1.onNext("🍐")
subject1.onNext("🍊")
subjectsSubject.onNext(subject2)
subject2.onNext("I would be ignored")
subject2.onNext("🐱")
subject1.onCompleted()
subject2.onNext("🐭")
}
//: [Next](@next) - [Table of Contents](Table_of_Contents)
| mit | 87f29c649564a89f7a2bfe87b1c3bb29 | 35.527778 | 273 | 0.677567 | 4.154818 | false | false | false | false |
SSamanta/SSQRScanner | SSQRCodeScanner/SSQRCodeScanner/SSQRScanner.swift | 1 | 2616 | //
// SSQRScanner.swift
//
// Created by Susim Samanta on 16/02/16.
// Copyright (c) 2015 Susim Samanta. All rights reserved.
//
import UIKit
import AVFoundation
public typealias SSQRScannerHandler = (_ obj : AnyObject? , _ error : NSError?) -> Void
public class SSQRScanner: NSObject,AVCaptureMetadataOutputObjectsDelegate {
var captureSession:AVCaptureSession?
var scannerPreviewLayer:AVCaptureVideoPreviewLayer?
var qrScannerHandler: SSQRScannerHandler?
public func createQRScannerOnCompletion(inView: UIView?, scannerHandler :@escaping SSQRScannerHandler) {
self.qrScannerHandler = scannerHandler
let captureDevice = AVCaptureDevice.default(for: AVMediaType.video)
var error:NSError?
let input: AnyObject!
do {
input = try AVCaptureDeviceInput(device: captureDevice!)
} catch let error1 as NSError {
error = error1
input = nil
}
if (error != nil) {
self.qrScannerHandler!(nil, error)
}
captureSession = AVCaptureSession()
captureSession?.addInput(input as! AVCaptureInput)
let captureMetadataOutput = AVCaptureMetadataOutput()
captureSession?.addOutput(captureMetadataOutput)
captureMetadataOutput.setMetadataObjectsDelegate(self, queue: DispatchQueue(label: "VideoDataOutputQueue"))
captureMetadataOutput.metadataObjectTypes = [AVMetadataObject.ObjectType.qr]
scannerPreviewLayer = AVCaptureVideoPreviewLayer(session: captureSession!)
scannerPreviewLayer?.videoGravity = AVLayerVideoGravity.resizeAspectFill
scannerPreviewLayer?.frame = inView!.layer.bounds
inView!.layer.addSublayer(scannerPreviewLayer!)
captureSession?.startRunning()
}
public func metadataOutput(_ output: AVCaptureMetadataOutput, didOutput metadataObjects: [AVMetadataObject], from connection: AVCaptureConnection) {
if metadataObjects.count == 0 {
DispatchQueue.main.async {
self.qrScannerHandler!(nil, nil)
}
}
let metadataObj = metadataObjects[0] as! AVMetadataMachineReadableCodeObject
if metadataObj.type == AVMetadataObject.ObjectType.qr {
_ = scannerPreviewLayer?.transformedMetadataObject(for: metadataObj as AVMetadataMachineReadableCodeObject) as! AVMetadataMachineReadableCodeObject
DispatchQueue.main.async {
self.qrScannerHandler!(metadataObj.stringValue as AnyObject, nil)
}
}
captureSession?.stopRunning()
}
}
| mit | a21ba78cea128787bb5f0afbe177385e | 40.52381 | 159 | 0.693807 | 5.686957 | false | false | false | false |
teaxus/TSAppEninge | Source/ThirdParty/SnapKit/ConstraintViewDSL.swift | 7 | 4710 | //
// SnapKit
//
// Copyright (c) 2011-Present SnapKit Team - https://github.com/SnapKit
//
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in
// all copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
// THE SOFTWARE.
#if os(iOS) || os(tvOS)
import UIKit
#else
import AppKit
#endif
public struct ConstraintViewDSL: ConstraintAttributesDSL {
@discardableResult
public func prepareConstraints(_ closure: (_ make: ConstraintMaker) -> Void) -> [Constraint] {
return ConstraintMaker.prepareConstraints(view: self.view, closure: closure)
}
public func makeConstraints(_ closure: (_ make: ConstraintMaker) -> Void) {
ConstraintMaker.makeConstraints(view: self.view, closure: closure)
}
public func remakeConstraints(_ closure: (_ make: ConstraintMaker) -> Void) {
ConstraintMaker.remakeConstraints(view: self.view, closure: closure)
}
public func updateConstraints(_ closure: (_ make: ConstraintMaker) -> Void) {
ConstraintMaker.updateConstraints(view: self.view, closure: closure)
}
public func removeConstraints() {
ConstraintMaker.removeConstraints(view: self.view)
}
public var contentHuggingHorizontalPriority: Float {
get {
return self.view.contentHuggingPriority(for: .horizontal)
}
set {
self.view.setContentHuggingPriority(newValue, for: .horizontal)
}
}
public var contentHuggingVerticalPriority: Float {
get {
return self.view.contentHuggingPriority(for: .vertical)
}
set {
self.view.setContentHuggingPriority(newValue, for: .vertical)
}
}
public var contentCompressionResistanceHorizontalPriority: Float {
get {
return self.view.contentCompressionResistancePriority(for: .horizontal)
}
set {
self.view.setContentHuggingPriority(newValue, for: .horizontal)
}
}
public var contentCompressionResistanceVerticalPriority: Float {
get {
return self.view.contentCompressionResistancePriority(for: .vertical)
}
set {
self.view.setContentCompressionResistancePriority(newValue, for: .vertical)
}
}
public var target: AnyObject? {
return self.view
}
internal let view: ConstraintView
internal init(view: ConstraintView) {
self.view = view
}
internal var layoutConstraints: [LayoutConstraint] {
return self.layoutConstraintsHashTable.allObjects
}
internal func add(layoutConstraints: [LayoutConstraint]) {
let hashTable = self.layoutConstraintsHashTable
for layoutConstraint in layoutConstraints {
hashTable.add(layoutConstraint)
}
}
internal func remove(layoutConstraints: [LayoutConstraint]) {
let hashTable = self.layoutConstraintsHashTable
for layoutConstraint in layoutConstraints {
hashTable.remove(layoutConstraint)
}
}
private var layoutConstraintsHashTable: NSHashTable<LayoutConstraint> {
let layoutConstraints: NSHashTable<LayoutConstraint>
if let existing = objc_getAssociatedObject(self.view, &layoutConstraintsKey) as? NSHashTable<LayoutConstraint> {
layoutConstraints = existing
} else {
layoutConstraints = NSHashTable<LayoutConstraint>.weakObjects()
objc_setAssociatedObject(self.view, &layoutConstraintsKey, layoutConstraints, .OBJC_ASSOCIATION_RETAIN_NONATOMIC)
}
return layoutConstraints
}
}
private var layoutConstraintsKey: UInt8 = 0
| mit | adfd224731aba2c4ed27b68afb129c58 | 33.888889 | 125 | 0.676008 | 5.244989 | false | false | false | false |
readium/r2-streamer-swift | r2-streamer-swiftTests/Parser/Audio/Services/AudioLocatorServiceTests.swift | 1 | 5750 | //
// Copyright 2020 Readium Foundation. All rights reserved.
// Use of this source code is governed by the BSD-style license
// available in the top-level LICENSE file of the project.
//
import Foundation
import XCTest
import R2Shared
@testable import R2Streamer
class AudioLocatorServiceTests: XCTestCase {
func testLocateLocatorMatchingReadingOrderHREF() {
let service = makeService(readingOrder: [
Link(href: "l1"),
Link(href: "l2")
])
let locator = Locator(href: "l1", type: "audio/mpeg", locations: .init(totalProgression: 0.53))
XCTAssertEqual(service.locate(locator), locator)
}
func testLocateLocatorReturnsNilIfNoMatch() {
let service = makeService(readingOrder: [
Link(href: "l1"),
Link(href: "l2")
])
let locator = Locator(href: "l3", type: "audio/mpeg", locations: .init(totalProgression: 0.53))
XCTAssertNil(service.locate(locator))
}
func testLocateLocatorUsesTotalProgression() {
let service = makeService(readingOrder: [
Link(href: "l1", type: "audio/mpeg", duration: 100),
Link(href: "l2", type: "audio/mpeg", duration: 100)
])
XCTAssertEqual(
service.locate(Locator(href: "wrong", type: "audio/mpeg", locations: .init(totalProgression: 0.49))),
Locator(href: "l1", type: "audio/mpeg", locations: .init(
fragments: ["t=98"],
progression: 98/100.0,
totalProgression: 0.49
))
)
XCTAssertEqual(
service.locate(Locator(href: "wrong", type: "audio/mpeg", locations: .init(totalProgression: 0.5))),
Locator(href: "l2", type: "audio/mpeg", locations: .init(
fragments: ["t=0"],
progression: 0,
totalProgression: 0.5
))
)
XCTAssertEqual(
service.locate(Locator(href: "wrong", type: "audio/mpeg", locations: .init(totalProgression: 0.51))),
Locator(href: "l2", type: "audio/mpeg", locations: .init(
fragments: ["t=2"],
progression: 0.02,
totalProgression: 0.51
))
)
}
func testLocateLocatorUsingTotalProgressionKeepsTitleAndText() {
let service = makeService(readingOrder: [
Link(href: "l1", type: "audio/mpeg", duration: 100),
Link(href: "l2", type: "audio/mpeg", duration: 100)
])
XCTAssertEqual(
service.locate(
Locator(
href: "wrong",
type: "wrong-type",
title: "Title",
locations: .init(
fragments: ["ignored"],
progression: 0.5,
totalProgression: 0.4,
position: 42,
otherLocations: ["other": "location"]
),
text: .init(after: "after", before: "before", highlight: "highlight")
)
),
Locator(
href: "l1",
type: "audio/mpeg",
title: "Title",
locations: .init(
fragments: ["t=80"],
progression: 80 / 100.0,
totalProgression: 0.4
),
text: .init(after: "after", before: "before", highlight: "highlight")
)
)
}
func testLocateProgression() {
let service = makeService(readingOrder: [
Link(href: "l1", type: "audio/mpeg", duration: 100),
Link(href: "l2", type: "audio/mpeg", duration: 100)
])
XCTAssertEqual(
service.locate(progression: 0),
Locator(href: "l1", type: "audio/mpeg", locations: .init(
fragments: ["t=0"],
progression: 0,
totalProgression: 0
))
)
XCTAssertEqual(
service.locate(progression: 0.49),
Locator(href: "l1", type: "audio/mpeg", locations: .init(
fragments: ["t=98"],
progression: 98/100.0,
totalProgression: 0.49
))
)
XCTAssertEqual(
service.locate(progression: 0.5),
Locator(href: "l2", type: "audio/mpeg", locations: .init(
fragments: ["t=0"],
progression: 0,
totalProgression: 0.5
))
)
XCTAssertEqual(
service.locate(progression: 0.51),
Locator(href: "l2", type: "audio/mpeg", locations: .init(
fragments: ["t=2"],
progression: 0.02,
totalProgression: 0.51
))
)
XCTAssertEqual(
service.locate(progression: 1),
Locator(href: "l2", type: "audio/mpeg", locations: .init(
fragments: ["t=100"],
progression: 1,
totalProgression: 1
))
)
}
func testLocateInvalidProgression() {
let service = makeService(readingOrder: [
Link(href: "l1", type: "audio/mpeg", duration: 100),
Link(href: "l2", type: "audio/mpeg", duration: 100)
])
XCTAssertNil(service.locate(progression: -0.5))
XCTAssertNil(service.locate(progression: 1.5))
}
private func makeService(readingOrder: [Link]) -> AudioLocatorService {
AudioLocatorService(
readingOrder: readingOrder
)
}
}
| bsd-3-clause | 699d5eb5986987f8fe570b73b877cded | 32.430233 | 113 | 0.497565 | 4.419677 | false | true | false | false |
kevinmbeaulieu/Signal-iOS | Signal/src/ViewControllers/AttachmentApprovalViewController.swift | 1 | 11998 | //
// Copyright (c) 2017 Open Whisper Systems. All rights reserved.
//
import Foundation
import MediaPlayer
class AttachmentApprovalViewController: UIViewController {
let TAG = "[AttachmentApprovalViewController]"
// MARK: Properties
let attachment: SignalAttachment
var successCompletion : (() -> Void)?
var videoPlayer: MPMoviePlayerController?
// MARK: Initializers
@available(*, unavailable, message:"use attachment: constructor instead.")
required init?(coder aDecoder: NSCoder) {
self.attachment = SignalAttachment.genericAttachment(data: nil,
dataUTI: kUTTypeContent as String,
filename:nil)
super.init(coder: aDecoder)
assertionFailure()
}
required init(attachment: SignalAttachment, successCompletion : @escaping () -> Void) {
assert(!attachment.hasError)
self.attachment = attachment
self.successCompletion = successCompletion
super.init(nibName: nil, bundle: nil)
}
// MARK: View Lifecycle
override func viewDidLoad() {
super.viewDidLoad()
view.backgroundColor = UIColor.black
self.navigationItem.leftBarButtonItem = UIBarButtonItem(barButtonSystemItem:.stop,
target:self,
action:#selector(donePressed))
self.navigationItem.title = NSLocalizedString("ATTACHMENT_APPROVAL_DIALOG_TITLE",
comment: "Title for the 'attachment approval' dialog.")
createViews()
}
override func viewWillAppear(_ animated: Bool) {
super.viewWillAppear(animated)
ViewControllerUtils.setAudioIgnoresHardwareMuteSwitch(true)
}
override func viewWillDisappear(_ animated: Bool) {
super.viewWillDisappear(animated)
ViewControllerUtils.setAudioIgnoresHardwareMuteSwitch(false)
}
// MARK: - Create Views
private func createViews() {
let previewTopMargin: CGFloat = 30
let previewHMargin: CGFloat = 20
let attachmentPreviewView = UIView()
self.view.addSubview(attachmentPreviewView)
attachmentPreviewView.autoPinWidthToSuperview(withMargin:previewHMargin)
attachmentPreviewView.autoPin(toTopLayoutGuideOf: self, withInset:previewTopMargin)
createButtonRow(attachmentPreviewView:attachmentPreviewView)
if attachment.isAnimatedImage {
createAnimatedPreview(attachmentPreviewView:attachmentPreviewView)
} else if attachment.isImage {
createImagePreview(attachmentPreviewView:attachmentPreviewView)
} else if attachment.isVideo {
createVideoPreview(attachmentPreviewView:attachmentPreviewView)
} else if attachment.isAudio {
createAudioPreview(attachmentPreviewView:attachmentPreviewView)
} else {
createGenericPreview(attachmentPreviewView:attachmentPreviewView)
}
}
private func createAudioPreview(attachmentPreviewView: UIView) {
// TODO: Add audio player.
createGenericPreview(attachmentPreviewView:attachmentPreviewView)
}
private func createAnimatedPreview(attachmentPreviewView: UIView) {
// Use Flipboard FLAnimatedImage library to display gifs
guard let animatedImage = FLAnimatedImage(gifData:attachment.data) else {
createGenericPreview(attachmentPreviewView:attachmentPreviewView)
return
}
let animatedImageView = FLAnimatedImageView()
animatedImageView.animatedImage = animatedImage
animatedImageView.contentMode = .scaleAspectFit
attachmentPreviewView.addSubview(animatedImageView)
animatedImageView.autoPinWidthToSuperview()
animatedImageView.autoPinHeightToSuperview()
}
private func createImagePreview(attachmentPreviewView: UIView) {
var image = attachment.image
if image == nil {
image = UIImage(data:attachment.data)
}
guard image != nil else {
createGenericPreview(attachmentPreviewView:attachmentPreviewView)
return
}
let imageView = UIImageView(image:image)
imageView.layer.minificationFilter = kCAFilterTrilinear
imageView.layer.magnificationFilter = kCAFilterTrilinear
imageView.contentMode = .scaleAspectFit
attachmentPreviewView.addSubview(imageView)
imageView.autoPinWidthToSuperview()
imageView.autoPinHeightToSuperview()
}
private func createVideoPreview(attachmentPreviewView: UIView) {
guard let dataUrl = attachment.getTemporaryDataUrl() else {
createGenericPreview(attachmentPreviewView:attachmentPreviewView)
return
}
guard let videoPlayer = MPMoviePlayerController(contentURL:dataUrl) else {
createGenericPreview(attachmentPreviewView:attachmentPreviewView)
return
}
videoPlayer.prepareToPlay()
videoPlayer.controlStyle = .default
videoPlayer.shouldAutoplay = false
attachmentPreviewView.addSubview(videoPlayer.view)
self.videoPlayer = videoPlayer
videoPlayer.view.autoPinWidthToSuperview()
videoPlayer.view.autoPinHeightToSuperview()
}
private func createGenericPreview(attachmentPreviewView: UIView) {
let stackView = UIView()
attachmentPreviewView.addSubview(stackView)
stackView.autoCenterInSuperview()
let imageSize = ScaleFromIPhone5To7Plus(175, 225)
let image = UIImage(named:"file-icon-large")
assert(image != nil)
let imageView = UIImageView(image:image)
imageView.layer.minificationFilter = kCAFilterTrilinear
imageView.layer.magnificationFilter = kCAFilterTrilinear
stackView.addSubview(imageView)
imageView.autoHCenterInSuperview()
imageView.autoPinEdge(toSuperviewEdge:.top)
imageView.autoSetDimension(.width, toSize:imageSize)
imageView.autoSetDimension(.height, toSize:imageSize)
var lastView: UIView = imageView
let labelFont = UIFont.ows_regularFont(withSize:ScaleFromIPhone5To7Plus(18, 24))
if let fileExtension = attachment.fileExtension {
let fileExtensionLabel = UILabel()
fileExtensionLabel.text = String(format:NSLocalizedString("ATTACHMENT_APPROVAL_FILE_EXTENSION_FORMAT",
comment: "Format string for file extension label in call interstitial view"),
fileExtension.capitalized)
fileExtensionLabel.textColor = UIColor.white
fileExtensionLabel.font = labelFont
fileExtensionLabel.textAlignment = .center
stackView.addSubview(fileExtensionLabel)
fileExtensionLabel.autoHCenterInSuperview()
fileExtensionLabel.autoPinEdge(.top, to:.bottom, of:lastView, withOffset:10)
lastView = fileExtensionLabel
}
let numberFormatter = NumberFormatter()
numberFormatter.numberStyle = NumberFormatter.Style.decimal
let fileSizeLabel = UILabel()
let fileSize = attachment.data.count
let kOneKilobyte = 1024
let kOneMegabyte = kOneKilobyte * kOneKilobyte
let fileSizeText = (fileSize > kOneMegabyte
? numberFormatter.string(from: NSNumber(value: fileSize / kOneMegabyte))! + " mb"
: (fileSize > kOneKilobyte
? numberFormatter.string(from: NSNumber(value: fileSize / kOneKilobyte))! + " kb"
: numberFormatter.string(from: NSNumber(value: fileSize))!))
fileSizeLabel.text = String(format:NSLocalizedString("ATTACHMENT_APPROVAL_FILE_SIZE_FORMAT",
comment: "Format string for file size label in call interstitial view. Embeds: {{file size as 'N mb' or 'N kb'}}."),
fileSizeText)
fileSizeLabel.textColor = UIColor.white
fileSizeLabel.font = labelFont
fileSizeLabel.textAlignment = .center
stackView.addSubview(fileSizeLabel)
fileSizeLabel.autoHCenterInSuperview()
fileSizeLabel.autoPinEdge(.top, to:.bottom, of:lastView, withOffset:10)
fileSizeLabel.autoPinEdge(toSuperviewEdge:.bottom)
}
private func createButtonRow(attachmentPreviewView: UIView) {
let buttonTopMargin = ScaleFromIPhone5To7Plus(30, 40)
let buttonBottomMargin = ScaleFromIPhone5To7Plus(25, 40)
let buttonHSpacing = ScaleFromIPhone5To7Plus(20, 30)
let buttonRow = UIView()
self.view.addSubview(buttonRow)
buttonRow.autoPinWidthToSuperview()
buttonRow.autoPinEdge(toSuperviewEdge:.bottom, withInset:buttonBottomMargin)
buttonRow.autoPinEdge(.top, to:.bottom, of:attachmentPreviewView, withOffset:buttonTopMargin)
// We use this invisible subview to ensure that the buttons are centered
// horizontally.
let buttonSpacer = UIView()
buttonRow.addSubview(buttonSpacer)
// Vertical positioning of this view doesn't matter.
buttonSpacer.autoPinEdge(toSuperviewEdge:.top)
buttonSpacer.autoSetDimension(.width, toSize:buttonHSpacing)
buttonSpacer.autoHCenterInSuperview()
let cancelButton = createButton(title: NSLocalizedString("TXT_CANCEL_TITLE",
comment: ""),
color : UIColor(rgbHex:0xff3B30),
action: #selector(cancelPressed))
buttonRow.addSubview(cancelButton)
cancelButton.autoPinEdge(toSuperviewEdge:.top)
cancelButton.autoPinEdge(toSuperviewEdge:.bottom)
cancelButton.autoPinEdge(.right, to:.left, of:buttonSpacer)
let sendButton = createButton(title: NSLocalizedString("ATTACHMENT_APPROVAL_SEND_BUTTON",
comment: "Label for 'send' button in the 'attachment approval' dialog."),
color : UIColor(rgbHex:0x4CD964),
action: #selector(sendPressed))
buttonRow.addSubview(sendButton)
sendButton.autoPinEdge(toSuperviewEdge:.top)
sendButton.autoPinEdge(toSuperviewEdge:.bottom)
sendButton.autoPinEdge(.left, to:.right, of:buttonSpacer)
}
private func createButton(title: String, color: UIColor, action: Selector) -> UIButton {
let buttonFont = UIFont.ows_mediumFont(withSize:ScaleFromIPhone5To7Plus(18, 22))
let buttonCornerRadius = ScaleFromIPhone5To7Plus(4, 5)
let buttonWidth = ScaleFromIPhone5To7Plus(110, 140)
let buttonHeight = ScaleFromIPhone5To7Plus(35, 45)
let button = UIButton()
button.setTitle(title, for:.normal)
button.setTitleColor(UIColor.white, for:.normal)
button.titleLabel!.font = buttonFont
button.backgroundColor = color
button.layer.cornerRadius = buttonCornerRadius
button.clipsToBounds = true
button.addTarget(self, action:action, for:.touchUpInside)
button.autoSetDimension(.width, toSize:buttonWidth)
button.autoSetDimension(.height, toSize:buttonHeight)
return button
}
// MARK: - Event Handlers
func donePressed(sender: UIButton) {
dismiss(animated: true, completion:nil)
}
func cancelPressed(sender: UIButton) {
dismiss(animated: true, completion:nil)
}
func sendPressed(sender: UIButton) {
let successCompletion = self.successCompletion
dismiss(animated: true, completion: {
successCompletion?()
})
}
}
| gpl-3.0 | aeb81531f4c72b12ad4b32e57d086b90 | 41.098246 | 177 | 0.657026 | 5.678183 | false | false | false | false |
Corotata/CRWeiBo | CRWeiBo/CRWeiBo/Classes/Modules/Main/Resource/CRNullViewController.swift | 1 | 3231 | //
// CRNullViewController.swift
// CRWeiBo
//
// Created by Corotata on 16/2/17.
// Copyright © 2016年 Corotata. All rights reserved.
//
import UIKit
class CRNullViewController: UITableViewController {
override func viewDidLoad() {
super.viewDidLoad()
// Uncomment the following line to preserve selection between presentations
// self.clearsSelectionOnViewWillAppear = false
// Uncomment the following line to display an Edit button in the navigation bar for this view controller.
// self.navigationItem.rightBarButtonItem = self.editButtonItem()
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
// MARK: - Table view data source
override func numberOfSectionsInTableView(tableView: UITableView) -> Int {
// #warning Incomplete implementation, return the number of sections
return 0
}
override func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
// #warning Incomplete implementation, return the number of rows
return 0
}
/*
override func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCellWithIdentifier("reuseIdentifier", forIndexPath: indexPath)
// Configure the cell...
return cell
}
*/
/*
// Override to support conditional editing of the table view.
override func tableView(tableView: UITableView, canEditRowAtIndexPath indexPath: NSIndexPath) -> Bool {
// Return false if you do not want the specified item to be editable.
return true
}
*/
/*
// Override to support editing the table view.
override func tableView(tableView: UITableView, commitEditingStyle editingStyle: UITableViewCellEditingStyle, forRowAtIndexPath indexPath: NSIndexPath) {
if editingStyle == .Delete {
// Delete the row from the data source
tableView.deleteRowsAtIndexPaths([indexPath], withRowAnimation: .Fade)
} else if editingStyle == .Insert {
// Create a new instance of the appropriate class, insert it into the array, and add a new row to the table view
}
}
*/
/*
// Override to support rearranging the table view.
override func tableView(tableView: UITableView, moveRowAtIndexPath fromIndexPath: NSIndexPath, toIndexPath: NSIndexPath) {
}
*/
/*
// Override to support conditional rearranging of the table view.
override func tableView(tableView: UITableView, canMoveRowAtIndexPath indexPath: NSIndexPath) -> Bool {
// Return false if you do not want the item to be re-orderable.
return true
}
*/
/*
// MARK: - Navigation
// In a storyboard-based application, you will often want to do a little preparation before navigation
override func prepareForSegue(segue: UIStoryboardSegue, sender: AnyObject?) {
// Get the new view controller using segue.destinationViewController.
// Pass the selected object to the new view controller.
}
*/
}
| mit | 6e886e1197b3ce37bdd5dab27ad62823 | 32.978947 | 157 | 0.686803 | 5.517949 | false | false | false | false |
TMTBO/TTAImagePickerController | TTAImagePickerController/Classes/Views/TTASelectCountLabel.swift | 1 | 1157 | //
// TTASelectCountLabel.swift
// Pods-TTAImagePickerController_Example
//
// Created by TobyoTenma on 02/07/2017.
//
import UIKit
class TTASelectCountLabel: UILabel {
var selectItemTintColor: UIColor? {
didSet {
guard selectItemTintColor != nil else { return }
backgroundColor = selectItemTintColor
}
}
override init(frame: CGRect) {
super.init(frame: frame)
setupUI()
}
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
override func layoutSubviews() {
super.layoutSubviews()
corner(with: bounds.height / 2)
}
}
extension TTASelectCountLabel {
func setupUI() {
backgroundColor = UIColor(red: 0, green: 122.0 / 255.0, blue: 1, alpha: 1)
textColor = .white
textAlignment = .center
adjustsFontSizeToFitWidth = true
}
func config(with count: Int) {
if count <= 0 {
isHidden = true
return
}
text = "\(count)"
isHidden = false
selectItemSpringAnimation()
}
}
| mit | 544b8ede822da242dfaf6f0b2499c0b9 | 21.686275 | 82 | 0.579948 | 4.609562 | false | false | false | false |
bitboylabs/selluv-ios | selluv-ios/selluv-ios/Classes/Controller/UI/Etc/SLVBankPickerView.swift | 1 | 4572 | //
// SLVBankPickerView.swift
// selluv-ios
//
// Created by 조백근 on 2017. 1. 12..
// Copyright © 2017년 BitBoy Labs. All rights reserved.
//
import UIKit
import SnapKit
import RealmSwift
//0.467
class SLVBankPickerView: UIView {
static let hRatio = CGFloat(1)
var config:STZPopupViewConfig?
var controller:UIViewController?
var collection: [AnyObject] = []
var propertyName: String = ""
var propertyCode: String = ""
var propertyRow: Int = -1
var isSellMode: Bool = false
@IBOutlet weak var sizePicker: UIPickerView!
@IBOutlet weak var sizeDoneButton: UIButton!
override func awakeFromNib() {
super.awakeFromNib()
self.sizePicker.delegate = self
self.sizePicker.dataSource = self
self.sizePicker.showsSelectionIndicator = true
self.setupLayout()
}
internal required init?(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)
}
override func layoutSubviews() {
super.layoutSubviews()
}
func setupLayout() {
let w = UIScreen.main.bounds.width
let h = UIScreen.main.bounds.height
self.frame = CGRect(x: 0, y: 0, width: w, height: h)
self.snp.makeConstraints { (make) in
make.width.equalTo(w)
make.height.equalTo(h)
}
}
func binding() {
let sname = TempInputInfoModel.shared.currentModel.accountbankName
let svalue = TempInputInfoModel.shared.currentModel.accountbankCode
self.binding(bname: sname, bvalue: svalue)
self.isSellMode = true
}
func binding(bname: String?, bvalue: String?) {
self.isSellMode = false
self.propertyRow = -1
let sname = bname
let svalue = bvalue
let list = CodeModel.shared.itemBank
for (i, v) in (list?.enumerated())! {
let code = v as Code
let name = code.name
let value = code.value
self.collection.append([name, value] as AnyObject)
if let nm = sname {
if let val = svalue {
if nm == name && val == value {
self.propertyRow = i
}
}
}
}
self.sizePicker.reloadAllComponents()
if self.propertyRow > -1 {
self.sizePicker.selectRow(self.propertyRow, inComponent: 0, animated: false)
}
}
func popBaseConstraints(config: STZPopupViewConfig, parent: UIViewController) {
self.config = config
self.controller = parent
self.translatesAutoresizingMaskIntoConstraints = false
}
func touchClose(sender: AnyObject) {
controller!.dismissPopupView()
}
@IBAction func touchDoneButton(_ sender: Any) {
if self.collection.count > self.propertyRow {
if self.propertyRow < 0 {
self.propertyRow = 0
}
let item = self.collection[self.propertyRow] as! [String]
let name = item.first
let value = item.last
if self.isSellMode == true {
TempInputInfoModel.shared.currentModel.accountbankName = name
TempInputInfoModel.shared.currentModel.accountbankCode = value
}
}
self.touchClose(sender: sender as AnyObject)
}
}
extension SLVBankPickerView: UIPickerViewDelegate {
func pickerView(_ pickerView: UIPickerView, didSelectRow row: Int, inComponent component: Int) {
if self.collection.count > row {
self.propertyRow = row
}
}
public func pickerView(_ pickerView: UIPickerView, attributedTitleForRow row: Int, forComponent component: Int) -> NSAttributedString? {
if self.collection.count > row {
let item = self.collection[row] as! [String]
let text = item.first
let myMutableString = NSMutableAttributedString(string: text!, attributes: [
NSFontAttributeName:UIFont.systemFont(ofSize: 16),
NSForegroundColorAttributeName: text_color_gold]
)
return myMutableString
}
return nil
}
}
extension SLVBankPickerView: UIPickerViewDataSource {
public func numberOfComponents(in pickerView: UIPickerView) -> Int {
return 1
}
public func pickerView(_ pickerView: UIPickerView, numberOfRowsInComponent component: Int) -> Int {
return self.collection.count
}
}
| mit | 900c89bbf806bc8e8e015717f36dc9c1 | 29.42 | 140 | 0.598948 | 4.704124 | false | false | false | false |
DianQK/LearnRxSwift | LearnRxSwift/Rx.playground/Pages/Observable.xcplaygroundpage/Contents.swift | 1 | 4062 | //: [上一节](@previous) - [目录](Index)
/*:
## 创建序列 Observable
> 基本上你不用担心会记不住这些操作符,我有一个好的建议,每当你想创建序列时,又忘记了都有什么方法,就试着想一下,你想创建什么样的序列,它应该有一个什么样的方法。
*/
import RxSwift
Observable<Int>.create { observer in
for i in 1...10 {
observer.onNext(i)
}
observer.onCompleted()
return NopDisposable.instance
}
.subscribe { event in
switch event {
case .Next(let value):
print(value)
case .Error(let error):
print(error)
case .Completed:
print("Completed")
}
}
/*:
### create
使用 Swift 闭包的方式创建序列,这里创建了一个 Just 的示例
*/
let myJust = { (singleElement: Int) -> Observable<Int> in
return Observable.create { observer in
observer.on(.Next(singleElement))
observer.on(.Completed)
return NopDisposable.instance
}
}
_ = myJust(5)
.subscribe { event in
print(event)
}
/*:
### `deferred`
只有在有观察者订阅时,才去创建序列

*/
let deferredSequence: Observable<Int> = Observable.deferred {
print("creating")
return Observable.create { observer in
print("emmiting")
observer.on(.Next(0))
observer.on(.Next(1))
observer.on(.Next(2))
return NopDisposable.instance
}
}
_ = deferredSequence
.subscribe { event in
print(event)
}
_ = deferredSequence
.subscribe { event in
print(event)
}
/*:
### empty
创建一个空的序列,只发射一个 `.Completed`
*/
let emptySequence = Observable<Int>.empty()
_ = emptySequence
.subscribe { event in
print(event)
}
/*:
### error
创建一个发射 error 终止的序列
*/
let error = NSError(domain: "Test", code: -1, userInfo: nil)
let erroredSequence = Observable<Int>.error(error)
_ = erroredSequence
.subscribe { event in
print(event)
}
/*:
### from
使用 SequenceType 创建序列
*/
let sequenceFromArray = [1, 2, 3, 4, 5].toObservable()
_ = sequenceFromArray
.subscribe { event in
print(event)
}
/*:
### interval
创建一个每隔一段时间就发射的递增序列
*/
let intervalSequence = Observable<Int>.interval(3, scheduler: MainScheduler.instance)
_ = intervalSequence.subscribe { event in
print(event)
}
/*:
### never
不创建序列,也不发送通知
*/
let neverSequence = Observable<Int>.never()
_ = neverSequence
.subscribe { _ in
print("这句话永远不会打出来.")
}
/*:
### just
只创建包含一个元素的序列。换言之,只发送一个值和 `.Completed`
*/
let singleElementSequence = Observable.just(32)
_ = singleElementSequence
.subscribe { event in
print(event)
}
/*:
### of
通过一组元素创建一个序列
*/
let sequenceOfElements/* : Observable<Int> */ = Observable.of(0, 1, 2, 3, 4, 5, 6, 7, 8, 9)
_ = sequenceOfElements
.subscribe { event in
print(event)
}
/*:
### range
创建一个有范围的递增序列
*/
let rangeSequence = Observable.range(start: 1, count: 10)
_ = rangeSequence.subscribe { event in
print(event)
}
/*:
### repeatElement
创建一个发射重复值的序列
*/
let repeatElementSequence = Observable.repeatElement(1)
//_ = repeatElementSequence.subscribe { event in
// print(event)
//}
// 请不要在 Playground 中随意运行这段无限执行的代码
/*:
### timer
创建一个带延迟的序列
*/
let timerSequence = Observable<Int>.timer(1, period: 1, scheduler: MainScheduler.instance)
_ = timerSequence.subscribe { event in
print(event)
}
/*:
看完了这么多的创建方式,然而并没有什么卵用,不过你可以思考以下这些序列可以或者可能用在什么场景中。
*/
//: [目录](@Index) - [下一节 >>](@next)
| mit | d326d24d1e6e08b836ac73d7692359b4 | 15.417476 | 96 | 0.635127 | 3.119926 | false | false | false | false |
macemmi/HBCI4Swift | HBCI4Swift/HBCI4Swift/Source/Sepa/HBCISepaUtility.swift | 1 | 1268 | //
// HBCISepaUtility.swift
// HBCI4Swift
//
// Created by Frank Emminghaus on 03.07.20.
// Copyright © 2020 Frank Emminghaus. All rights reserved.
//
import Foundation
class HBCISepaUtility {
let numberFormatter = NumberFormatter();
let dateFormatter = DateFormatter();
init() {
initFormatters();
}
fileprivate func initFormatters() {
numberFormatter.decimalSeparator = ".";
numberFormatter.alwaysShowsDecimalSeparator = true;
numberFormatter.minimumFractionDigits = 2;
numberFormatter.maximumFractionDigits = 2;
numberFormatter.generatesDecimalNumbers = true;
dateFormatter.dateFormat = "yyyy-MM-dd";
}
func stringToNumber(_ s:String) ->NSDecimalNumber? {
if let number = numberFormatter.number(from: s) as? NSDecimalNumber {
return number;
} else {
logInfo("Sepa document parser: not able to convert \(s) to a value");
return nil;
}
}
func stringToDate(_ s:String) ->Date? {
if let date = dateFormatter.date(from: s) {
return date;
} else {
logInfo("Sepa document parser: not able to convert \(s) to a date");
return nil;
}
}
}
| gpl-2.0 | 85e3705bad336c23a8c4f7d8d7e1212e | 25.957447 | 81 | 0.611681 | 4.710037 | false | false | false | false |
brave/browser-ios | brave/src/frontend/sync/SyncSelectDeviceTypeViewController.swift | 1 | 5318 | /* This Source Code Form is subject to the terms of the Mozilla Public License, v. 2.0. If a copy of the MPL was not distributed with this file, You can obtain one at http://mozilla.org/MPL/2.0/. */
import UIKit
import Shared
import SnapKit
import pop
class SyncDeviceTypeButton: UIControl {
var imageView: UIImageView = UIImageView()
var label: UILabel = UILabel()
var type: DeviceType!
var pressed: Bool = false {
didSet {
if pressed {
label.textColor = BraveUX.BraveOrange
if let anim = POPSpringAnimation(propertyNamed: kPOPLayerScaleXY) {
anim.toValue = NSValue(cgSize: CGSize(width: 0.9, height: 0.9))
layer.pop_add(anim, forKey: "size")
}
}
else {
label.textColor = BraveUX.GreyJ
if let anim = POPSpringAnimation(propertyNamed: kPOPLayerScaleXY) {
anim.toValue = NSValue(cgSize: CGSize(width: 1.0, height: 1.0))
layer.pop_add(anim, forKey: "size")
}
}
}
}
convenience init(image: String, title: String, type: DeviceType) {
self.init(frame: CGRect.zero)
clipsToBounds = false
backgroundColor = UIColor.white
layer.cornerRadius = 12
layer.shadowColor = BraveUX.GreyJ.cgColor
layer.shadowRadius = 3
layer.shadowOpacity = 0.1
layer.shadowOffset = CGSize(width: 0, height: 1)
imageView.image = UIImage(named: image)
imageView.contentMode = .center
imageView.tintColor = BraveUX.GreyJ
addSubview(imageView)
label.text = title
label.font = UIFont.systemFont(ofSize: 17.0, weight: UIFont.Weight.bold)
label.textColor = BraveUX.GreyJ
label.textAlignment = .center
addSubview(label)
self.type = type
imageView.snp.makeConstraints { (make) in
make.centerX.equalTo(self)
make.centerY.equalTo(self).offset(-20)
}
label.snp.makeConstraints { (make) in
make.top.equalTo(imageView.snp.bottom).offset(20)
make.centerX.equalTo(self)
make.width.equalTo(self)
}
// Prevents bug where user can tap on two device types at once.
isExclusiveTouch = true
}
override init(frame: CGRect) {
super.init(frame: frame)
}
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
override func beginTracking(_ touch: UITouch, with event: UIEvent?) -> Bool {
pressed = true
return true
}
override func endTracking(_ touch: UITouch?, with event: UIEvent?) {
pressed = false
}
override func cancelTracking(with event: UIEvent?) {
pressed = false
}
}
class SyncSelectDeviceTypeViewController: SyncViewController {
var syncInitHandler: ((String, DeviceType) -> ())?
let loadingView = UIView()
let mobileButton = SyncDeviceTypeButton(image: "sync-mobile", title: Strings.SyncAddMobileButton, type: .mobile)
let computerButton = SyncDeviceTypeButton(image: "sync-computer", title: Strings.SyncAddComputerButton, type: .computer)
override func viewDidLoad() {
super.viewDidLoad()
title = Strings.SyncAddDevice
let stackView = UIStackView()
stackView.axis = .vertical
stackView.distribution = .fillEqually
stackView.spacing = 16
view.addSubview(stackView)
stackView.snp.makeConstraints { make in
make.top.equalTo(self.topLayoutGuide.snp.bottom).offset(16)
make.left.right.equalTo(self.view).inset(16)
make.bottom.equalTo(self.view.safeArea.bottom).inset(16)
}
stackView.addArrangedSubview(mobileButton)
stackView.addArrangedSubview(computerButton)
mobileButton.addTarget(self, action: #selector(addDevice), for: .touchUpInside)
computerButton.addTarget(self, action: #selector(addDevice), for: .touchUpInside)
// Loading View
// This should be general, and abstracted
let spinner = UIActivityIndicatorView(activityIndicatorStyle: .whiteLarge)
spinner.startAnimating()
loadingView.backgroundColor = UIColor(white: 0.5, alpha: 0.5)
loadingView.isHidden = true
loadingView.addSubview(spinner)
view.addSubview(loadingView)
spinner.snp.makeConstraints { (make) in
make.center.equalTo(spinner.superview!)
}
loadingView.snp.makeConstraints { (make) in
make.edges.equalTo(loadingView.superview!)
}
}
@objc func addDevice(sender: SyncDeviceTypeButton) {
doIfConnected {
self.syncInitHandler?(sender.label.text ?? "", sender.type)
}
}
}
extension SyncSelectDeviceTypeViewController: NavigationPrevention {
func enableNavigationPrevention() {
navigationItem.hidesBackButton = true
loadingView.isHidden = false
}
func disableNavigationPrevention() {
navigationItem.hidesBackButton = false
loadingView.isHidden = true
}
}
| mpl-2.0 | f4daf6d5d91915968eaeef1dafca27fa | 32.031056 | 198 | 0.620158 | 4.773788 | false | false | false | false |
cohix/SPBannerView | SPBannerView/CEMKit.swift | 1 | 47069 | //
// CEMKit.swift
// CEMKit-Swift
//
// Created by Cem Olcay on 05/11/14.
// Copyright (c) 2014 Cem Olcay. All rights reserved.
//
import Foundation
import UIKit
// MARK: - AppDelegate
// let APPDELEGATE: AppDelegate = UIApplication.sharedApplication().delegate as AppDelegate
// MARK: - UIView
let UIViewAnimationDuration: NSTimeInterval = 1
let UIViewAnimationSpringDamping: CGFloat = 0.5
let UIViewAnimationSpringVelocity: CGFloat = 0.5
extension UIView {
// MARK: Custom Initilizer
convenience init (x: CGFloat,
y: CGFloat,
w: CGFloat,
h: CGFloat) {
self.init (frame: CGRect (x: x, y: y, width: w, height: h))
}
convenience init (superView: UIView) {
self.init (frame: CGRect (origin: CGPointZero, size: superView.size))
}
}
// MARK: Frame Extensions
extension UIView {
var x: CGFloat {
get {
return self.frame.origin.x
} set (value) {
self.frame = CGRect (x: value, y: self.y, width: self.w, height: self.h)
}
}
var y: CGFloat {
get {
return self.frame.origin.y
} set (value) {
self.frame = CGRect (x: self.x, y: value, width: self.w, height: self.h)
}
}
var w: CGFloat {
get {
return self.frame.size.width
} set (value) {
self.frame = CGRect (x: self.x, y: self.y, width: value, height: self.h)
}
}
var h: CGFloat {
get {
return self.frame.size.height
} set (value) {
self.frame = CGRect (x: self.x, y: self.y, width: self.w, height: value)
}
}
var left: CGFloat {
get {
return self.x
} set (value) {
self.x = value
}
}
var right: CGFloat {
get {
return self.x + self.w
} set (value) {
self.x = value - self.w
}
}
var top: CGFloat {
get {
return self.y
} set (value) {
self.y = value
}
}
var bottom: CGFloat {
get {
return self.y + self.h
} set (value) {
self.y = value - self.h
}
}
var position: CGPoint {
get {
return self.frame.origin
} set (value) {
self.frame = CGRect (origin: value, size: self.frame.size)
}
}
var size: CGSize {
get {
return self.frame.size
} set (value) {
self.frame = CGRect (origin: self.frame.origin, size: value)
}
}
func leftWithOffset (offset: CGFloat) -> CGFloat {
return self.left - offset
}
func rightWithOffset (offset: CGFloat) -> CGFloat {
return self.right + offset
}
func topWithOffset (offset: CGFloat) -> CGFloat {
return self.top - offset
}
func bottomWithOffset (offset: CGFloat) -> CGFloat {
return self.bottom + offset
}
}
// MARK: Transform Extensions
extension UIView {
func setRotationX (x: CGFloat) {
var transform = CATransform3DIdentity
transform.m34 = 1.0 / -1000.0
transform = CATransform3DRotate(transform, degreesToRadians(x), 1.0, 0.0, 0.0)
self.layer.transform = transform
}
func setRotationY (y: CGFloat) {
var transform = CATransform3DIdentity
transform.m34 = 1.0 / -1000.0
transform = CATransform3DRotate(transform, degreesToRadians(y), 0.0, 1.0, 0.0)
self.layer.transform = transform
}
func setRotationZ (z: CGFloat) {
var transform = CATransform3DIdentity
transform.m34 = 1.0 / -1000.0
transform = CATransform3DRotate(transform, degreesToRadians(z), 0.0, 0.0, 1.0)
self.layer.transform = transform
}
func setRotation (
x: CGFloat,
y: CGFloat,
z: CGFloat) {
var transform = CATransform3DIdentity
transform.m34 = 1.0 / -1000.0
transform = CATransform3DRotate(transform, degreesToRadians(x), 1.0, 0.0, 0.0)
transform = CATransform3DRotate(transform, degreesToRadians(y), 0.0, 1.0, 0.0)
transform = CATransform3DRotate(transform, degreesToRadians(z), 0.0, 0.0, 1.0)
self.layer.transform = transform
}
func setScale (
x: CGFloat,
y: CGFloat) {
var transform = CATransform3DIdentity
transform.m34 = 1.0 / -1000.0
transform = CATransform3DScale(transform, x, y, 1)
self.layer.transform = transform
}
}
// MARK: Layer Extensions
extension UIView {
func setAnchorPosition (anchorPosition: AnchorPosition) {
println(anchorPosition.rawValue)
self.layer.anchorPoint = anchorPosition.rawValue
}
func setCornerRadius (radius: CGFloat) {
self.layer.cornerRadius = radius
self.layer.masksToBounds = true
}
func addShadow (
offset: CGSize,
radius: CGFloat,
color: UIColor,
opacity: Float,
cornerRadius: CGFloat? = nil) {
self.layer.shadowOffset = offset
self.layer.shadowRadius = radius
self.layer.shadowOpacity = opacity
self.layer.shadowColor = color.CGColor
if let r = cornerRadius {
self.layer.shadowPath = UIBezierPath(roundedRect: bounds, cornerRadius: r).CGPath
}
}
func addBorder (
width: CGFloat,
color: UIColor) {
self.layer.borderWidth = width
self.layer.borderColor = color.CGColor
self.layer.masksToBounds = true
}
func drawCircle (
fillColor: UIColor,
strokeColor: UIColor,
strokeWidth: CGFloat) {
let path = UIBezierPath (roundedRect: CGRect (x: 0, y: 0, width: self.w, height: self.w), cornerRadius: self.w/2)
let shapeLayer = CAShapeLayer ()
shapeLayer.path = path.CGPath
shapeLayer.fillColor = fillColor.CGColor
shapeLayer.strokeColor = strokeColor.CGColor
shapeLayer.lineWidth = strokeWidth
self.layer.addSublayer(shapeLayer)
}
func drawStroke (
width: CGFloat,
color: UIColor) {
let path = UIBezierPath (roundedRect: CGRect (x: 0, y: 0, width: self.w, height: self.w), cornerRadius: self.w/2)
let shapeLayer = CAShapeLayer ()
shapeLayer.path = path.CGPath
shapeLayer.fillColor = UIColor.clearColor().CGColor
shapeLayer.strokeColor = color.CGColor
shapeLayer.lineWidth = width
self.layer.addSublayer(shapeLayer)
}
func drawArc (
from: CGFloat,
to: CGFloat,
clockwise: Bool,
width: CGFloat,
fillColor: UIColor,
strokeColor: UIColor,
lineCap: String) {
let path = UIBezierPath (arcCenter: self.center, radius: self.w/2, startAngle: degreesToRadians(from), endAngle: degreesToRadians(to), clockwise: clockwise)
let shapeLayer = CAShapeLayer ()
shapeLayer.path = path.CGPath
shapeLayer.fillColor = fillColor.CGColor
shapeLayer.strokeColor = strokeColor.CGColor
shapeLayer.lineWidth = width
self.layer.addSublayer(shapeLayer)
}
}
// MARK: Animation Extensions
extension UIView {
func spring (
animations: (()->Void),
completion: ((Bool)->Void)? = nil) {
spring(UIViewAnimationDuration,
animations: animations,
completion: completion)
}
func spring (
duration: NSTimeInterval,
animations: (()->Void),
completion: ((Bool)->Void)? = nil) {
UIView.animateWithDuration(UIViewAnimationDuration,
delay: 0,
usingSpringWithDamping: UIViewAnimationSpringDamping,
initialSpringVelocity: UIViewAnimationSpringVelocity,
options: UIViewAnimationOptions.AllowAnimatedContent,
animations: animations,
completion: completion)
}
func animate (
duration: NSTimeInterval,
animations: (()->Void),
completion: ((Bool)->Void)? = nil) {
UIView.animateWithDuration(duration,
animations: animations,
completion: completion)
}
func animate (
animations: (()->Void),
completion: ((Bool)->Void)? = nil) {
animate(
UIViewAnimationDuration,
animations: animations,
completion: completion)
}
func pop () {
setScale(1.1, y: 1.1)
spring(0.2, animations: { [unowned self] () -> Void in
self.setScale(1, y: 1)
})
}
}
// MARK: Render Extensions
extension UIView {
func toImage () -> UIImage {
UIGraphicsBeginImageContextWithOptions(bounds.size, opaque, 0.0)
drawViewHierarchyInRect(bounds, afterScreenUpdates: false)
let img = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
return img
}
}
// MARK: Gesture Extensions
extension UIView {
func addTapGesture (
tapNumber: Int,
target: AnyObject, action: Selector) {
let tap = UITapGestureRecognizer (target: target, action: action)
tap.numberOfTapsRequired = tapNumber
addGestureRecognizer(tap)
userInteractionEnabled = true
}
func addTapGesture (
tapNumber: Int,
action: ((UITapGestureRecognizer)->())?) {
let tap = BlockTap (tapCount: tapNumber,
fingerCount: 1,
action: action)
addGestureRecognizer(tap)
userInteractionEnabled = true
}
func addSwipeGesture (
direction: UISwipeGestureRecognizerDirection,
numberOfTouches: Int,
target: AnyObject,
action: Selector) {
let swipe = UISwipeGestureRecognizer (target: target, action: action)
swipe.direction = direction
swipe.numberOfTouchesRequired = numberOfTouches
addGestureRecognizer(swipe)
userInteractionEnabled = true
}
func addSwipeGesture (
direction: UISwipeGestureRecognizerDirection,
numberOfTouches: Int,
action: ((UISwipeGestureRecognizer)->())?) {
let swipe = BlockSwipe (direction: direction,
fingerCount: numberOfTouches,
action: action)
addGestureRecognizer(swipe)
userInteractionEnabled = true
}
func addPanGesture (
target: AnyObject,
action: Selector) {
let pan = UIPanGestureRecognizer (target: target, action: action)
addGestureRecognizer(pan)
userInteractionEnabled = true
}
func addPanGesture (action: ((UIPanGestureRecognizer)->())?) {
let pan = BlockPan (action: action)
addGestureRecognizer(pan)
userInteractionEnabled = true
}
func addPinchGesture (
target: AnyObject,
action: Selector) {
let pinch = UIPinchGestureRecognizer (target: target, action: action)
addGestureRecognizer(pinch)
userInteractionEnabled = true
}
func addPinchGesture (action: ((UIPinchGestureRecognizer)->())?) {
let pinch = BlockPinch (action: action)
addGestureRecognizer(pinch)
userInteractionEnabled = true
}
func addLongPressGesture (
target: AnyObject,
action: Selector) {
let longPress = UILongPressGestureRecognizer (target: target, action: action)
addGestureRecognizer(longPress)
userInteractionEnabled = true
}
func addLongPressGesture (action: ((UILongPressGestureRecognizer)->())?) {
let longPress = BlockLongPress (action: action)
addGestureRecognizer(longPress)
userInteractionEnabled = true
}
}
// MARK: - UIScrollView
extension UIScrollView {
var contentHeight: CGFloat {
get {
return contentSize.height
} set (value) {
contentSize = CGSize (width: contentSize.width, height: value)
}
}
var contentWidth: CGFloat {
get {
return contentSize.height
} set (value) {
contentSize = CGSize (width: value, height: contentSize.height)
}
}
var offsetX: CGFloat {
get {
return contentOffset.x
} set (value) {
contentOffset = CGPoint (x: value, y: contentOffset.y)
}
}
var offsetY: CGFloat {
get {
return contentOffset.y
} set (value) {
contentOffset = CGPoint (x: contentOffset.x, y: value)
}
}
}
// MARK: - UIViewController
extension UIViewController {
var top: CGFloat {
get {
if let me = self as? UINavigationController {
return me.visibleViewController.top
}
if let nav = self.navigationController {
if nav.navigationBarHidden {
return view.top
} else {
return nav.navigationBar.bottom
}
} else {
return view.top
}
}
}
var bottom: CGFloat {
get {
if let me = self as? UINavigationController {
return me.visibleViewController.bottom
}
if let tab = tabBarController {
if tab.tabBar.hidden {
return view.bottom
} else {
return tab.tabBar.top
}
} else {
return view.bottom
}
}
}
var tabBarHeight: CGFloat {
get {
if let me = self as? UINavigationController {
return me.visibleViewController.tabBarHeight
}
if let tab = self.tabBarController {
return tab.tabBar.frame.size.height
}
return 0
}
}
var navigationBarHeight: CGFloat {
get {
if let me = self as? UINavigationController {
return me.visibleViewController.navigationBarHeight
}
if let nav = self.navigationController {
return nav.navigationBar.h
}
return 0
}
}
var navigationBarColor: UIColor? {
get {
if let me = self as? UINavigationController {
return me.visibleViewController.navigationBarColor
}
return navigationController?.navigationBar.tintColor
} set (value) {
navigationController?.navigationBar.barTintColor = value
}
}
var navBar: UINavigationBar? {
get {
return navigationController?.navigationBar
}
}
var applicationFrame: CGRect {
get {
return CGRect (x: view.x, y: top, width: view.w, height: bottom - top)
}
}
func push (vc: UIViewController) {
navigationController?.pushViewController(vc, animated: true)
}
func pop () {
navigationController?.popViewControllerAnimated(true)
}
func present (vc: UIViewController) {
presentViewController(vc, animated: true, completion: nil)
}
func dismiss (completion: (()->Void)?) {
dismissViewControllerAnimated(true, completion: completion)
}
}
// MARK: - UILabel
private var UILabelAttributedStringArray: UInt8 = 0
extension UILabel {
var attributedStrings: [NSAttributedString]? {
get {
return objc_getAssociatedObject(self, &UILabelAttributedStringArray) as? [NSAttributedString]
} set (value) {
objc_setAssociatedObject(self, &UILabelAttributedStringArray, value, UInt(OBJC_ASSOCIATION_RETAIN_NONATOMIC))
}
}
func addAttributedString (
text: String,
color: UIColor,
font: UIFont) {
var att = NSAttributedString (text: text, color: color, font: font)
self.addAttributedString(att)
}
func addAttributedString (attributedString: NSAttributedString) {
var att: NSMutableAttributedString?
if let a = self.attributedText {
att = NSMutableAttributedString (attributedString: a)
att?.appendAttributedString(attributedString)
} else {
att = NSMutableAttributedString (attributedString: attributedString)
attributedStrings = []
}
attributedStrings?.append(attributedString)
self.attributedText = NSAttributedString (attributedString: att!)
}
func updateAttributedStringAtIndex (index: Int,
attributedString: NSAttributedString) {
if let att = attributedStrings?[index] {
attributedStrings?.removeAtIndex(index)
attributedStrings?.insert(attributedString, atIndex: index)
let updated = NSMutableAttributedString ()
for att in attributedStrings! {
updated.appendAttributedString(att)
}
self.attributedText = NSAttributedString (attributedString: updated)
}
}
func updateAttributedStringAtIndex (index: Int,
newText: String) {
if let att = attributedStrings?[index] {
let newAtt = NSMutableAttributedString (string: newText)
att.enumerateAttributesInRange(NSMakeRange(0, count(att.string)-1),
options: NSAttributedStringEnumerationOptions.LongestEffectiveRangeNotRequired,
usingBlock: { (attribute, range, stop) -> Void in
for (key, value) in attribute {
newAtt.addAttribute(key as! String, value: value, range: range)
}
}
)
updateAttributedStringAtIndex(index, attributedString: newAtt)
}
}
func getEstimatedSize (
width: CGFloat = CGFloat.max,
height: CGFloat = CGFloat.max) -> CGSize {
return sizeThatFits(CGSize(width: width, height: height))
}
func getEstimatedHeight () -> CGFloat {
return sizeThatFits(CGSize(width: w, height: CGFloat.max)).height
}
func getEstimatedWidth () -> CGFloat {
return sizeThatFits(CGSize(width: CGFloat.max, height: h)).width
}
func fitHeight () {
self.h = getEstimatedHeight()
}
func fitWidth () {
self.w = getEstimatedWidth()
}
func fitSize () {
self.fitWidth()
self.fitHeight()
sizeToFit()
}
// Text, TextColor, TextAlignment, Font
convenience init (
frame: CGRect,
text: String,
textColor: UIColor,
textAlignment: NSTextAlignment,
font: UIFont) {
self.init(frame: frame)
self.text = text
self.textColor = textColor
self.textAlignment = textAlignment
self.font = font
self.numberOfLines = 0
}
convenience init (
x: CGFloat,
y: CGFloat,
width: CGFloat,
height: CGFloat,
text: String,
textColor: UIColor,
textAlignment: NSTextAlignment,
font: UIFont) {
self.init(frame: CGRect (x: x, y: y, width: width, height: height))
self.text = text
self.textColor = textColor
self.textAlignment = textAlignment
self.font = font
self.numberOfLines = 0
}
convenience init (
x: CGFloat,
y: CGFloat,
width: CGFloat,
text: String,
textColor: UIColor,
textAlignment: NSTextAlignment,
font: UIFont) {
self.init(frame: CGRect (x: x, y: y, width: width, height: 10.0))
self.text = text
self.textColor = textColor
self.textAlignment = textAlignment
self.font = font
self.numberOfLines = 0
self.fitHeight()
}
convenience init (
x: CGFloat,
y: CGFloat,
width: CGFloat,
padding: CGFloat,
text: String,
textColor: UIColor,
textAlignment: NSTextAlignment,
font: UIFont) {
self.init(frame: CGRect (x: x, y: y, width: width, height: 10.0))
self.text = text
self.textColor = textColor
self.textAlignment = textAlignment
self.font = font
self.numberOfLines = 0
self.h = self.getEstimatedHeight() + 2*padding
}
convenience init (
x: CGFloat,
y: CGFloat,
text: String,
textColor: UIColor,
textAlignment: NSTextAlignment,
font: UIFont) {
self.init(frame: CGRect (x: x, y: y, width: 10.0, height: 10.0))
self.text = text
self.textColor = textColor
self.textAlignment = textAlignment
self.font = font
self.numberOfLines = 0
self.fitSize()
}
// AttributedText
convenience init (
frame: CGRect,
attributedText: NSAttributedString,
textAlignment: NSTextAlignment) {
self.init(frame: frame)
self.attributedText = attributedText
self.textAlignment = textAlignment
self.numberOfLines = 0
}
convenience init (
x: CGFloat,
y: CGFloat,
width: CGFloat,
height: CGFloat,
attributedText: NSAttributedString,
textAlignment: NSTextAlignment) {
self.init(frame: CGRect (x: x, y: y, width: width, height: height))
self.attributedText = attributedText
self.textAlignment = textAlignment
self.numberOfLines = 0
}
convenience init (
x: CGFloat,
y: CGFloat,
width: CGFloat,
attributedText: NSAttributedString,
textAlignment: NSTextAlignment) {
self.init(frame: CGRect (x: x, y: y, width: width, height: 10.0))
self.attributedText = attributedText
self.textAlignment = textAlignment
self.numberOfLines = 0
self.fitHeight()
}
convenience init (
x: CGFloat,
y: CGFloat,
width: CGFloat,
padding: CGFloat,
attributedText: NSAttributedString,
textAlignment: NSTextAlignment) {
self.init(frame: CGRect (x: x, y: y, width: width, height: 10.0))
self.attributedText = attributedText
self.textAlignment = textAlignment
self.numberOfLines = 0
self.fitHeight()
self.h += padding*2
}
convenience init (
x: CGFloat,
y: CGFloat,
attributedText: NSAttributedString,
textAlignment: NSTextAlignment) {
self.init(frame: CGRect (x: x, y: y, width: 10.0, height: 10.0))
self.attributedText = attributedText
self.textAlignment = textAlignment
self.numberOfLines = 0
self.fitSize()
}
}
// MARK: NSAttributedString
extension NSAttributedString {
enum NSAttributedStringStyle {
case plain
case underline (NSUnderlineStyle, UIColor)
case strike (UIColor, CGFloat)
func attribute () -> [NSString: NSObject] {
switch self {
case .plain:
return [:]
case .underline(let styleName, let color):
return [NSUnderlineStyleAttributeName: styleName.rawValue, NSUnderlineColorAttributeName: color]
case .strike(let color, let width):
return [NSStrikethroughColorAttributeName: color, NSStrikethroughStyleAttributeName: width]
}
}
}
func addAtt (attribute: [NSString: NSObject]) -> NSAttributedString {
let mutable = NSMutableAttributedString (attributedString: self)
let c = count(string)
for (key, value) in attribute {
mutable.addAttribute(key as! String, value: value, range: NSMakeRange(0, c))
}
return mutable
}
func addStyle (style: NSAttributedStringStyle) -> NSAttributedString {
return addAtt(style.attribute())
}
convenience init (
text: String,
color: UIColor,
font: UIFont,
style: NSAttributedStringStyle = .plain) {
var atts = [NSFontAttributeName as NSString: font, NSForegroundColorAttributeName as NSString: color]
atts += style.attribute()
self.init (string: text, attributes: atts)
}
convenience init (image: UIImage) {
let att = NSTextAttachment ()
att.image = image
self.init (attachment: att)
}
class func withAttributedStrings (mutableString: (NSMutableAttributedString)->()) -> NSAttributedString {
var mutable = NSMutableAttributedString ()
mutableString (mutable)
return mutable
}
}
// MARK: - String
extension String {
subscript (i: Int) -> String {
return String(Array(self)[i])
}
}
// MARK: - UIFont
enum FontType: String {
case Regular = "Regular"
case Bold = "Bold"
case DemiBold = "DemiBold"
case Light = "Light"
case UltraLight = "UltraLight"
case Italic = "Italic"
case Thin = "Thin"
case Book = "Book"
case Roman = "Roman"
case Medium = "Medium"
case MediumItalic = "MediumItalic"
case CondensedMedium = "CondensedMedium"
case CondensedExtraBold = "CondensedExtraBold"
case SemiBold = "SemiBold"
case BoldItalic = "BoldItalic"
case Heavy = "Heavy"
}
enum FontName: String {
case HelveticaNeue = "HelveticaNeue"
case Helvetica = "Helvetica"
case Futura = "Futura"
case Menlo = "Menlo"
case Avenir = "Avenir"
case AvenirNext = "AvenirNext"
case Didot = "Didot"
case AmericanTypewriter = "AmericanTypewriter"
case Baskerville = "Baskerville"
case Geneva = "Geneva"
case GillSans = "GillSans"
case SanFranciscoDisplay = "SanFranciscoDisplay"
case Seravek = "Seravek"
}
extension UIFont {
class func PrintFontFamily (font: FontName) {
let arr = UIFont.fontNamesForFamilyName(font.rawValue)
for name in arr {
println(name)
}
}
class func Font (
name: FontName,
type: FontType,
size: CGFloat) -> UIFont {
return UIFont (name: name.rawValue + "-" + type.rawValue, size: size)!
}
class func HelveticaNeue (
type: FontType,
size: CGFloat) -> UIFont {
return Font(.HelveticaNeue, type: type, size: size)
}
class func AvenirNext (
type: FontType,
size: CGFloat) -> UIFont {
return UIFont.Font(.AvenirNext, type: type, size: size)
}
class func AvenirNextDemiBold (size: CGFloat) -> UIFont {
return AvenirNext(.DemiBold, size: size)
}
class func AvenirNextRegular (size: CGFloat) -> UIFont {
return AvenirNext(.Regular, size: size)
}
}
// MARK: - UIImageView
extension UIImageView {
convenience init (
frame: CGRect,
imageName: String) {
self.init (frame: frame, image: UIImage (named: imageName)!)
}
convenience init (
frame: CGRect,
image: UIImage) {
self.init (frame: frame)
self.image = image
self.contentMode = .ScaleAspectFit
}
convenience init (
x: CGFloat,
y: CGFloat,
width: CGFloat,
image: UIImage) {
self.init (frame: CGRect (x: x, y: y, width: width, height: image.aspectHeightForWidth(width)), image: image)
}
convenience init (
x: CGFloat,
y: CGFloat,
height: CGFloat,
image: UIImage) {
self.init (frame: CGRect (x: x, y: y, width: image.aspectWidthForHeight(height), height: height), image: image)
}
func imageWithUrl (url: String) {
imageRequest(url, { (image) -> Void in
if let img = image {
self.image = image
}
})
}
func imageWithUrl (url: String, placeholder: UIImage) {
self.image = placeholder
imageRequest(url, { (image) -> Void in
if let img = image {
self.image = image
}
})
}
func imageWithUrl (url: String, placeholderNamed: String) {
self.image = UIImage (named: placeholderNamed)
imageRequest(url, { (image) -> Void in
if let img = image {
self.image = image
}
})
}
}
// MARK: - UIImage
extension UIImage {
func aspectResizeWithWidth (width: CGFloat) -> UIImage {
let aspectSize = CGSize (width: width, height: aspectHeightForWidth(width))
UIGraphicsBeginImageContext(aspectSize)
self.drawInRect(CGRect(origin: CGPointZero, size: aspectSize))
let img = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
return img
}
func aspectResizeWithHeight (height: CGFloat) -> UIImage {
let aspectSize = CGSize (width: aspectWidthForHeight(height), height: height)
UIGraphicsBeginImageContext(aspectSize)
self.drawInRect(CGRect(origin: CGPointZero, size: aspectSize))
let img = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
return img
}
func aspectHeightForWidth (width: CGFloat) -> CGFloat {
return (width * self.size.height) / self.size.width
}
func aspectWidthForHeight (height: CGFloat) -> CGFloat {
return (height * self.size.width) / self.size.height
}
}
// MARK: - UIColor
extension UIColor {
class func randomColor () -> UIColor {
var randomRed:CGFloat = CGFloat(drand48())
var randomGreen:CGFloat = CGFloat(drand48())
var randomBlue:CGFloat = CGFloat(drand48())
return UIColor(red: randomRed,
green: randomGreen,
blue: randomBlue,
alpha: 1.0)
}
class func RGBColor (
r: CGFloat,
g: CGFloat,
b: CGFloat) -> UIColor {
return UIColor (red: r / 255.0,
green: g / 255.0,
blue: b / 255.0,
alpha: 1)
}
class func RGBAColor (
r: CGFloat,
g: CGFloat,
b: CGFloat,
a: CGFloat) -> UIColor {
return UIColor (red: r / 255.0,
green: g / 255.0,
blue: b / 255.0,
alpha: a)
}
class func BarTintRGBColor (
r: CGFloat,
g: CGFloat,
b: CGFloat) -> UIColor {
return UIColor (red: (r / 255.0) - 0.12,
green: (g / 255.0) - 0.12,
blue: (b / 255.0) - 0.12,
alpha: 1)
}
class func Gray (gray: CGFloat) -> UIColor {
return self.RGBColor(gray, g: gray, b: gray)
}
class func Gray (gray: CGFloat, alpha: CGFloat) -> UIColor {
return self.RGBAColor(gray, g: gray, b: gray, a: alpha)
}
class func HexColor (hex: String) -> UIColor {
var red: CGFloat = 0.0
var green: CGFloat = 0.0
var blue: CGFloat = 0.0
var alpha: CGFloat = 1.0
var rgba = hex
if !rgba.hasPrefix("#") {
rgba = "#" + rgba
}
let index = advance(rgba.startIndex, 1)
let hex = rgba.substringFromIndex(index)
let scanner = NSScanner(string: hex)
var hexValue: CUnsignedLongLong = 0
if scanner.scanHexLongLong(&hexValue) {
switch (count(hex)) {
case 3:
red = CGFloat((hexValue & 0xF00) >> 8) / 15.0
green = CGFloat((hexValue & 0x0F0) >> 4) / 15.0
blue = CGFloat(hexValue & 0x00F) / 15.0
case 4:
red = CGFloat((hexValue & 0xF000) >> 12) / 15.0
green = CGFloat((hexValue & 0x0F00) >> 8) / 15.0
blue = CGFloat((hexValue & 0x00F0) >> 4) / 15.0
alpha = CGFloat(hexValue & 0x000F) / 15.0
case 6:
red = CGFloat((hexValue & 0xFF0000) >> 16) / 255.0
green = CGFloat((hexValue & 0x00FF00) >> 8) / 255.0
blue = CGFloat(hexValue & 0x0000FF) / 255.0
case 8:
red = CGFloat((hexValue & 0xFF000000) >> 24) / 255.0
green = CGFloat((hexValue & 0x00FF0000) >> 16) / 255.0
blue = CGFloat((hexValue & 0x0000FF00) >> 8) / 255.0
alpha = CGFloat(hexValue & 0x000000FF) / 255.0
default:
print("Invalid RGB string, number of characters after '#' should be either 3, 4, 6 or 8")
}
} else {
println("Scan hex error")
}
return UIColor (red: red, green:green, blue:blue, alpha:alpha)
}
}
// MARK - UIScreen
extension UIScreen {
class var Orientation: UIInterfaceOrientation {
get {
return UIApplication.sharedApplication().statusBarOrientation
}
}
class var ScreenWidth: CGFloat {
get {
if UIInterfaceOrientationIsPortrait(Orientation) {
return UIScreen.mainScreen().bounds.size.width
} else {
return UIScreen.mainScreen().bounds.size.height
}
}
}
class var ScreenHeight: CGFloat {
get {
if UIInterfaceOrientationIsPortrait(Orientation) {
return UIScreen.mainScreen().bounds.size.height
} else {
return UIScreen.mainScreen().bounds.size.width
}
}
}
class var StatusBarHeight: CGFloat {
get {
return UIApplication.sharedApplication().statusBarFrame.height
}
}
}
// MARK: - Array
extension Array {
mutating func removeObject<U: Equatable> (object: U) {
var index: Int?
for (idx, objectToCompare) in enumerate(self) {
if let to = objectToCompare as? U {
if object == to {
index = idx
}
}
}
if(index != nil) {
self.removeAtIndex(index!)
}
}
}
// MARK: - CGSize
func + (left: CGSize, right: CGSize) -> CGSize {
return CGSize (width: left.width + right.width, height: left.height + right.height)
}
func - (left: CGSize, right: CGSize) -> CGSize {
return CGSize (width: left.width - right.width, height: left.width - right.width)
}
// MARK: - CGPoint
func + (left: CGPoint, right: CGPoint) -> CGPoint {
return CGPoint (x: left.x + right.x, y: left.y + right.y)
}
func - (left: CGPoint, right: CGPoint) -> CGPoint {
return CGPoint (x: left.x - right.x, y: left.y - right.y)
}
enum AnchorPosition: CGPoint {
case TopLeft = "{0, 0}"
case TopCenter = "{0.5, 0}"
case TopRight = "{1, 0}"
case MidLeft = "{0, 0.5}"
case MidCenter = "{0.5, 0.5}"
case MidRight = "{1, 0.5}"
case BottomLeft = "{0, 1}"
case BottomCenter = "{0.5, 1}"
case BottomRight = "{1, 1}"
}
extension CGPoint: StringLiteralConvertible {
public init(stringLiteral value: StringLiteralType) {
self = CGPointFromString(value)
}
public init(extendedGraphemeClusterLiteral value: StringLiteralType) {
self = CGPointFromString(value)
}
public init(unicodeScalarLiteral value: StringLiteralType) {
self = CGPointFromString(value)
}
}
// MARK: - CGFloat
func degreesToRadians (angle: CGFloat) -> CGFloat {
return (CGFloat (M_PI) * angle) / 180.0
}
func normalizeValue (
value: CGFloat,
min: CGFloat,
max: CGFloat) -> CGFloat {
return (max - min) / value
}
func convertNormalizedValue (
normalizedValue: CGFloat,
min: CGFloat,
max: CGFloat) -> CGFloat {
return ((max - min) * normalizedValue) + min
}
func clamp (
value: CGFloat,
minimum: CGFloat,
maximum: CGFloat) -> CGFloat {
return min (maximum, max(value, minimum))
}
func aspectHeightForTargetAspectWidth (
currentHeight: CGFloat,
currentWidth: CGFloat,
targetAspectWidth: CGFloat) -> CGFloat {
return (targetAspectWidth * currentHeight) / currentWidth
}
func aspectWidthForTargetAspectHeight (
currentHeight: CGFloat,
currentWidth: CGFloat,
targetAspectHeight: CGFloat) -> CGFloat {
return (targetAspectHeight * currentWidth) / currentHeight
}
// MARK: - Dictionary
func += <KeyType, ValueType> (inout left: Dictionary<KeyType, ValueType>,
right: Dictionary<KeyType, ValueType>) {
for (k, v) in right {
left.updateValue(v, forKey: k)
}
}
// MARK: - Dispatch
func delay (
seconds: Double,
queue: dispatch_queue_t = dispatch_get_main_queue(),
after: ()->()) {
let time = dispatch_time(DISPATCH_TIME_NOW, Int64(seconds * Double(NSEC_PER_SEC)))
dispatch_after(time, queue, after)
}
// MARK: - DownloadTask
func urlRequest (
url: String,
success: (NSData?)->Void,
error: ((NSError)->Void)? = nil) {
NSURLConnection.sendAsynchronousRequest(
NSURLRequest (URL: NSURL (string: url)!),
queue: NSOperationQueue.mainQueue(),
completionHandler: { response, data, err in
if let e = err {
error? (e)
} else {
success (data)
}
})
}
func imageRequest (
url: String,
success: (UIImage?)->Void) {
urlRequest(url, { (data) -> Void in
if let d = data {
success (UIImage (data: d))
}
})
}
func jsonRequest (
url: String,
success: (AnyObject?->Void),
error: ((NSError)->Void)?) {
urlRequest(
url,
{ (data)->Void in
let json: AnyObject? = dataToJsonDict(data)
success (json)
},
error: { (err)->Void in
if let e = error {
e (err)
}
})
}
func dataToJsonDict (data: NSData?) -> AnyObject? {
if let d = data {
var error: NSError?
let json: AnyObject? = NSJSONSerialization.JSONObjectWithData(
d,
options: NSJSONReadingOptions.AllowFragments,
error: &error)
if let e = error {
return nil
} else {
return json
}
} else {
return nil
}
}
// MARK: - UIAlertController
func alert (
title: String,
message: String,
cancelAction: ((UIAlertAction!)->Void)? = nil,
okAction: ((UIAlertAction!)->Void)? = nil) -> UIAlertController {
let a = UIAlertController (title: title, message: message, preferredStyle: .Alert)
if let ok = okAction {
a.addAction(UIAlertAction(title: "OK", style: .Default, handler: ok))
a.addAction(UIAlertAction(title: "Cancel", style: .Cancel, handler: cancelAction))
} else {
a.addAction(UIAlertAction(title: "OK", style: .Cancel, handler: cancelAction))
}
return a
}
func actionSheet (
title: String,
message: String,
actions: [UIAlertAction]) -> UIAlertController {
let a = UIAlertController (title: title, message: message, preferredStyle: .ActionSheet)
for action in actions {
a.addAction(action)
}
return a
}
// MARK: - UIBarButtonItem
func barButtonItem (
imageName: String,
size: CGFloat,
action: (AnyObject)->()) -> UIBarButtonItem {
let button = BlockButton (frame: CGRect(x: 0, y: 0, width: size, height: size))
button.setImage(UIImage(named: imageName), forState: .Normal)
button.actionBlock = action
return UIBarButtonItem (customView: button)
}
func barButtonItem (
imageName: String,
action: (AnyObject)->()) -> UIBarButtonItem {
return barButtonItem(imageName, 20, action)
}
func barButtonItem (
title: String,
color: UIColor,
action: (AnyObject)->()) -> UIBarButtonItem {
let button = BlockButton (frame: CGRect(x: 0, y: 0, width: 20, height: 20))
button.setTitle(title, forState: .Normal)
button.setTitleColor(color, forState: .Normal)
button.actionBlock = action
button.sizeToFit()
return UIBarButtonItem (customView: button)
}
// MARK: - BlockButton
class BlockButton: UIButton {
init (x: CGFloat, y: CGFloat, w: CGFloat, h: CGFloat) {
super.init (frame: CGRect (x: x, y: y, width: w, height: h))
}
override init (frame: CGRect) {
super.init(frame: frame)
}
required init(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)
}
var actionBlock: ((sender: BlockButton) -> ())? {
didSet {
self.addTarget(self, action: "action:", forControlEvents: UIControlEvents.TouchUpInside)
}
}
func action (sender: BlockButton) {
actionBlock! (sender: sender)
}
}
// MARK: - BlockWebView
class BlockWebView: UIWebView, UIWebViewDelegate {
var didStartLoad: ((NSURLRequest) -> ())?
var didFinishLoad: ((NSURLRequest) -> ())?
var didFailLoad: ((NSURLRequest, NSError) -> ())?
var shouldStartLoadingRequest: ((NSURLRequest) -> (Bool))?
override init(frame: CGRect) {
super.init(frame: frame)
delegate = self
}
required init(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)
}
func webViewDidStartLoad(webView: UIWebView) {
didStartLoad? (webView.request!)
}
func webViewDidFinishLoad(webView: UIWebView) {
didFinishLoad? (webView.request!)
}
func webView(
webView: UIWebView,
didFailLoadWithError error: NSError) {
didFailLoad? (webView.request!, error)
}
func webView(
webView: UIWebView,
shouldStartLoadWithRequest request: NSURLRequest,
navigationType: UIWebViewNavigationType) -> Bool {
if let should = shouldStartLoadingRequest {
return should (request)
} else {
return true
}
}
}
// MARK: BlockTap
class BlockTap: UITapGestureRecognizer {
private var tapAction: ((UITapGestureRecognizer) -> Void)?
override init(target: AnyObject, action: Selector) {
super.init(target: target, action: action)
}
convenience init (
tapCount: Int,
fingerCount: Int,
action: ((UITapGestureRecognizer) -> Void)?) {
self.init()
self.numberOfTapsRequired = tapCount
self.numberOfTouchesRequired = fingerCount
self.tapAction = action
self.addTarget(self, action: "didTap:")
}
func didTap (tap: UITapGestureRecognizer) {
tapAction? (tap)
}
}
// MARK: BlockPan
class BlockPan: UIPanGestureRecognizer {
private var panAction: ((UIPanGestureRecognizer) -> Void)?
override init(target: AnyObject, action: Selector) {
super.init(target: target, action: action)
}
convenience init (action: ((UIPanGestureRecognizer) -> Void)?) {
self.init()
self.panAction = action
self.addTarget(self, action: "didPan:")
}
func didPan (pan: UIPanGestureRecognizer) {
panAction? (pan)
}
}
// MARK: BlockSwipe
class BlockSwipe: UISwipeGestureRecognizer {
private var swipeAction: ((UISwipeGestureRecognizer) -> Void)?
override init(target: AnyObject, action: Selector) {
super.init(target: target, action: action)
}
convenience init (direction: UISwipeGestureRecognizerDirection,
fingerCount: Int,
action: ((UISwipeGestureRecognizer) -> Void)?) {
self.init()
self.direction = direction
numberOfTouchesRequired = fingerCount
swipeAction = action
addTarget(self, action: "didSwipe:")
}
func didSwipe (swipe: UISwipeGestureRecognizer) {
swipeAction? (swipe)
}
}
// MARK: BlockPinch
class BlockPinch: UIPinchGestureRecognizer {
private var pinchAction: ((UIPinchGestureRecognizer) -> Void)?
override init(target: AnyObject, action: Selector) {
super.init(target: target, action: action)
}
convenience init (action: ((UIPinchGestureRecognizer) -> Void)?) {
self.init()
pinchAction = action
addTarget(self, action: "didPinch:")
}
func didPinch (pinch: UIPinchGestureRecognizer) {
pinchAction? (pinch)
}
}
// MARK: BlockLongPress
class BlockLongPress: UILongPressGestureRecognizer {
private var longPressAction: ((UILongPressGestureRecognizer) -> Void)?
override init(target: AnyObject, action: Selector) {
super.init(target: target, action: action)
}
convenience init (action: ((UILongPressGestureRecognizer) -> Void)?) {
self.init()
longPressAction = action
addTarget(self, action: "didLongPressed:")
}
func didLongPressed (longPress: UILongPressGestureRecognizer) {
longPressAction? (longPress)
}
}
| mit | 2239f27e8e20cbcc880d56bb1206ecbd | 25.758954 | 168 | 0.556311 | 4.876606 | false | false | false | false |
Syject/MyLessPass-iOS | LessPass/API/Options.swift | 1 | 1021 | //
// Options.swift
// LessPass
//
// Created by Dan Slupskiy on 11.01.17.
// Copyright © 2017 Syject. All rights reserved.
//
import Foundation
import SwiftyJSON
class Options: NSObject {
var site = ""
var login = ""
var hasLowerCaseLetters = true
var hasUpperCaseLetters = true
var hasNumbers = true
var hasSymbols = true
var length = 16
var counter = 1
var version = 2
}
@objc class SavedOption: Options {
var id: String?
override init() {
super.init()
}
init(with json:JSON) {
id = json["id"].string!
super.init()
counter = json["counter"].int!
length = json["length"].int!
login = json["login"].string!
hasLowerCaseLetters = json["lowercase"].bool!
hasUpperCaseLetters = json["uppercase"].bool!
hasNumbers = json["numbers"].bool!
hasSymbols = json["symbols"].bool!
site = json["site"].string!
version = json["version"].int!
}
}
| gpl-3.0 | adbd23a2b94e591b0766a1fde8fd7ec1 | 21.173913 | 53 | 0.577451 | 4.031621 | false | false | false | false |
everald/JetPack | Sources/Extensions/MapKit/MKCoordinateSpan.swift | 2 | 222 | import MapKit
extension MKCoordinateSpan: Equatable {
public static func == (a: MKCoordinateSpan, b: MKCoordinateSpan) -> Bool {
return a.latitudeDelta == b.latitudeDelta && a.longitudeDelta == b.longitudeDelta
}
}
| mit | 032e39f502e2a0169e65bedf34b9c166 | 23.666667 | 83 | 0.738739 | 4.352941 | false | false | false | false |
rsmoz/swift-corelibs-foundation | Foundation/NSCharacterSet.swift | 1 | 11732 | // This source file is part of the Swift.org open source project
//
// Copyright (c) 2014 - 2015 Apple Inc. and the Swift project authors
// Licensed under Apache License v2.0 with Runtime Library Exception
//
// See http://swift.org/LICENSE.txt for license information
// See http://swift.org/CONTRIBUTORS.txt for the list of Swift project authors
//
import CoreFoundation
#if os(OSX) || os(iOS)
let kCFCharacterSetControl = CFCharacterSetPredefinedSet.Control
let kCFCharacterSetWhitespace = CFCharacterSetPredefinedSet.Whitespace
let kCFCharacterSetWhitespaceAndNewline = CFCharacterSetPredefinedSet.WhitespaceAndNewline
let kCFCharacterSetDecimalDigit = CFCharacterSetPredefinedSet.DecimalDigit
let kCFCharacterSetLetter = CFCharacterSetPredefinedSet.Letter
let kCFCharacterSetLowercaseLetter = CFCharacterSetPredefinedSet.LowercaseLetter
let kCFCharacterSetUppercaseLetter = CFCharacterSetPredefinedSet.UppercaseLetter
let kCFCharacterSetNonBase = CFCharacterSetPredefinedSet.NonBase
let kCFCharacterSetDecomposable = CFCharacterSetPredefinedSet.Decomposable
let kCFCharacterSetAlphaNumeric = CFCharacterSetPredefinedSet.AlphaNumeric
let kCFCharacterSetPunctuation = CFCharacterSetPredefinedSet.Punctuation
let kCFCharacterSetCapitalizedLetter = CFCharacterSetPredefinedSet.CapitalizedLetter
let kCFCharacterSetSymbol = CFCharacterSetPredefinedSet.Symbol
let kCFCharacterSetNewline = CFCharacterSetPredefinedSet.Newline
let kCFCharacterSetIllegal = CFCharacterSetPredefinedSet.Illegal
#endif
public class NSCharacterSet : NSObject, NSCopying, NSMutableCopying, NSCoding {
typealias CFType = CFCharacterSetRef
private var _base = _CFInfo(typeID: CFCharacterSetGetTypeID())
private var _hashValue = CFHashCode(0)
private var _buffer = UnsafeMutablePointer<Void>()
private var _length = CFIndex(0)
private var _annex = UnsafeMutablePointer<Void>()
internal var _cfObject: CFType {
return unsafeBitCast(self, CFType.self)
}
internal var _cfMutableObject: CFMutableCharacterSetRef {
return unsafeBitCast(self, CFMutableCharacterSetRef.self)
}
public override var hash: Int {
get {
return Int(bitPattern: CFHash(_cfObject))
}
}
public override func isEqual(object: AnyObject?) -> Bool {
if let cs = object as? NSCharacterSet {
return CFEqual(_cfObject, cs._cfObject)
} else {
return false
}
}
public override var description: String {
get {
return CFCopyDescription(_cfObject)._swiftObject
}
}
deinit {
_CFDeinit(self)
}
public class func controlCharacterSet() -> NSCharacterSet {
return CFCharacterSetGetPredefined(kCFCharacterSetControl)._nsObject
}
public class func whitespaceCharacterSet() -> NSCharacterSet {
return CFCharacterSetGetPredefined(kCFCharacterSetWhitespace)._nsObject
}
public class func whitespaceAndNewlineCharacterSet() -> NSCharacterSet {
return CFCharacterSetGetPredefined(kCFCharacterSetWhitespaceAndNewline)._nsObject
}
public class func decimalDigitCharacterSet() -> NSCharacterSet {
return CFCharacterSetGetPredefined(kCFCharacterSetDecimalDigit)._nsObject
}
public class func letterCharacterSet() -> NSCharacterSet {
return CFCharacterSetGetPredefined(kCFCharacterSetLetter)._nsObject
}
public class func lowercaseLetterCharacterSet() -> NSCharacterSet {
return CFCharacterSetGetPredefined(kCFCharacterSetLowercaseLetter)._nsObject
}
public class func uppercaseLetterCharacterSet() -> NSCharacterSet {
return CFCharacterSetGetPredefined(kCFCharacterSetUppercaseLetter)._nsObject
}
public class func nonBaseCharacterSet() -> NSCharacterSet {
return CFCharacterSetGetPredefined(kCFCharacterSetNonBase)._nsObject
}
public class func alphanumericCharacterSet() -> NSCharacterSet {
return CFCharacterSetGetPredefined(kCFCharacterSetAlphaNumeric)._nsObject
}
public class func decomposableCharacterSet() -> NSCharacterSet {
return CFCharacterSetGetPredefined(kCFCharacterSetDecomposable)._nsObject
}
public class func illegalCharacterSet() -> NSCharacterSet {
return CFCharacterSetGetPredefined(kCFCharacterSetIllegal)._nsObject
}
public class func punctuationCharacterSet() -> NSCharacterSet {
return CFCharacterSetGetPredefined(kCFCharacterSetPunctuation)._nsObject
}
public class func capitalizedLetterCharacterSet() -> NSCharacterSet {
return CFCharacterSetGetPredefined(kCFCharacterSetCapitalizedLetter)._nsObject
}
public class func symbolCharacterSet() -> NSCharacterSet {
return CFCharacterSetGetPredefined(kCFCharacterSetSymbol)._nsObject
}
public class func newlineCharacterSet() -> NSCharacterSet {
return CFCharacterSetGetPredefined(kCFCharacterSetNewline)._nsObject
}
public init(range aRange: NSRange) {
super.init()
_CFCharacterSetInitWithCharactersInRange(_cfMutableObject, CFRangeMake(aRange.location, aRange.length))
}
public init(charactersInString aString: String) {
super.init()
_CFCharacterSetInitWithCharactersInString(_cfMutableObject, aString._cfObject)
}
public init(bitmapRepresentation data: NSData) {
super.init()
_CFCharacterSetInitWithBitmapRepresentation(_cfMutableObject, data._cfObject)
}
public convenience init?(contentsOfFile fName: String) {
if let data = NSData(contentsOfFile: fName) {
self.init(bitmapRepresentation: data)
} else {
return nil
}
}
public convenience required init(coder aDecoder: NSCoder) {
self.init(charactersInString: "")
}
public func characterIsMember(aCharacter: unichar) -> Bool {
return CFCharacterSetIsCharacterMember(_cfObject, UniChar(aCharacter))
}
public var bitmapRepresentation: NSData {
get {
return CFCharacterSetCreateBitmapRepresentation(kCFAllocatorSystemDefault, _cfObject)._nsObject
}
}
public var invertedSet: NSCharacterSet {
get {
return CFCharacterSetCreateInvertedSet(kCFAllocatorSystemDefault, _cfObject)._nsObject
}
}
public func longCharacterIsMember(theLongChar: UTF32Char) -> Bool {
return CFCharacterSetIsLongCharacterMember(_cfObject, theLongChar)
}
public func isSupersetOfSet(theOtherSet: NSCharacterSet) -> Bool {
return CFCharacterSetIsSupersetOfSet(_cfObject, theOtherSet._cfObject)
}
public func hasMemberInPlane(thePlane: UInt8) -> Bool {
return CFCharacterSetHasMemberInPlane(_cfObject, CFIndex(thePlane))
}
public override func copy() -> AnyObject {
return copyWithZone(nil)
}
public func copyWithZone(zone: NSZone) -> AnyObject {
return CFCharacterSetCreateCopy(kCFAllocatorSystemDefault, self._cfObject)
}
public override func mutableCopy() -> AnyObject {
return mutableCopyWithZone(nil)
}
public func mutableCopyWithZone(zone: NSZone) -> AnyObject {
return CFCharacterSetCreateMutableCopy(kCFAllocatorSystemDefault, _cfObject)._nsObject
}
public func encodeWithCoder(aCoder: NSCoder) {
}
}
public class NSMutableCharacterSet : NSCharacterSet {
public convenience required init(coder aDecoder: NSCoder) {
NSUnimplemented()
}
public func addCharactersInRange(aRange: NSRange) {
CFCharacterSetAddCharactersInRange(_cfMutableObject , CFRangeMake(aRange.location, aRange.length))
}
public func removeCharactersInRange(aRange: NSRange) {
CFCharacterSetRemoveCharactersInRange(_cfMutableObject , CFRangeMake(aRange.location, aRange.length))
}
public func addCharactersInString(aString: String) {
CFCharacterSetAddCharactersInString(_cfMutableObject, aString._cfObject)
}
public func removeCharactersInString(aString: String) {
CFCharacterSetRemoveCharactersInString(_cfMutableObject, aString._cfObject)
}
public func formUnionWithCharacterSet(otherSet: NSCharacterSet) {
CFCharacterSetUnion(_cfMutableObject, otherSet._cfObject)
}
public func formIntersectionWithCharacterSet(otherSet: NSCharacterSet) {
CFCharacterSetIntersect(_cfMutableObject, otherSet._cfObject)
}
public func invert() {
CFCharacterSetInvert(_cfMutableObject)
}
public override class func controlCharacterSet() -> NSMutableCharacterSet {
return NSCharacterSet.controlCharacterSet().mutableCopy() as! NSMutableCharacterSet
}
public override class func whitespaceCharacterSet() -> NSMutableCharacterSet {
return NSCharacterSet.whitespaceCharacterSet().mutableCopy() as! NSMutableCharacterSet
}
public override class func whitespaceAndNewlineCharacterSet() -> NSMutableCharacterSet {
return NSCharacterSet.whitespaceAndNewlineCharacterSet().mutableCopy() as! NSMutableCharacterSet
}
public override class func decimalDigitCharacterSet() -> NSMutableCharacterSet {
return NSCharacterSet.decimalDigitCharacterSet().mutableCopy() as! NSMutableCharacterSet
}
public override class func letterCharacterSet() -> NSMutableCharacterSet {
return NSCharacterSet.letterCharacterSet().mutableCopy() as! NSMutableCharacterSet
}
public override class func lowercaseLetterCharacterSet() -> NSMutableCharacterSet {
return NSCharacterSet.lowercaseLetterCharacterSet().mutableCopy() as! NSMutableCharacterSet
}
public override class func uppercaseLetterCharacterSet() -> NSMutableCharacterSet {
return NSCharacterSet.uppercaseLetterCharacterSet().mutableCopy() as! NSMutableCharacterSet
}
public override class func nonBaseCharacterSet() -> NSMutableCharacterSet {
return NSCharacterSet.nonBaseCharacterSet().mutableCopy() as! NSMutableCharacterSet
}
public override class func alphanumericCharacterSet() -> NSMutableCharacterSet {
return NSCharacterSet.alphanumericCharacterSet().mutableCopy() as! NSMutableCharacterSet
}
public override class func decomposableCharacterSet() -> NSMutableCharacterSet {
return NSCharacterSet.decomposableCharacterSet().mutableCopy() as! NSMutableCharacterSet
}
public override class func illegalCharacterSet() -> NSMutableCharacterSet {
return NSCharacterSet.illegalCharacterSet().mutableCopy() as! NSMutableCharacterSet
}
public override class func punctuationCharacterSet() -> NSMutableCharacterSet {
return NSCharacterSet.punctuationCharacterSet().mutableCopy() as! NSMutableCharacterSet
}
public override class func capitalizedLetterCharacterSet() -> NSMutableCharacterSet {
return NSCharacterSet.capitalizedLetterCharacterSet().mutableCopy() as! NSMutableCharacterSet
}
public override class func symbolCharacterSet() -> NSMutableCharacterSet {
return NSCharacterSet.symbolCharacterSet().mutableCopy() as! NSMutableCharacterSet
}
public override class func newlineCharacterSet() -> NSMutableCharacterSet {
return NSCharacterSet.newlineCharacterSet().mutableCopy() as! NSMutableCharacterSet
}
}
extension CFCharacterSetRef : _NSBridgable {
typealias NSType = NSCharacterSet
internal var _nsObject: NSType {
return unsafeBitCast(self, NSType.self)
}
}
| apache-2.0 | d6c8f3c4f764c354bdd91831fe749edf | 37.090909 | 111 | 0.732782 | 6.414434 | false | false | false | false |
tlax/looper | looper/Controller/Camera/CCamera.swift | 1 | 5933 | import UIKit
class CCamera:CController
{
weak var viewCamera:VCamera!
private var refreshCamera:Bool
private let kAfterShoot:TimeInterval = 0.6
override init()
{
refreshCamera = false
super.init()
}
required init?(coder:NSCoder)
{
return nil
}
deinit
{
NotificationCenter.default.removeObserver(self)
}
override func viewDidLoad()
{
super.viewDidLoad()
if MSession.sharedInstance.camera == nil
{
MSession.sharedInstance.camera = MCamera()
}
NotificationCenter.default.addObserver(
self,
selector:#selector(notifiedCameraLoading(sender:)),
name:Notification.cameraLoading,
object:nil)
NotificationCenter.default.addObserver(
self,
selector:#selector(notifiedCameraLoadFinished(sender:)),
name:Notification.cameraLoadFinished,
object:nil)
guard
let model:MCamera = MSession.sharedInstance.camera
else
{
return
}
if model.records.isEmpty
{
DispatchQueue.main.asyncAfter(
deadline:DispatchTime.now() + kAfterShoot)
{ [weak self] in
self?.refreshCamera = true
self?.shoot()
}
}
else
{
refreshCamera = true
viewCamera.refresh()
}
}
override func viewDidAppear(_ animated:Bool)
{
super.viewDidAppear(animated)
if refreshCamera
{
viewCamera.refresh()
}
}
override func loadView()
{
let viewCamera:VCamera = VCamera(controller:self)
self.viewCamera = viewCamera
view = viewCamera
}
//MARK: notifications
func notifiedCameraLoading(sender notification:Notification)
{
DispatchQueue.main.async
{ [weak self] in
self?.viewCamera.showLoading()
}
}
func notifiedCameraLoadFinished(sender notification:Notification)
{
DispatchQueue.main.async
{ [weak self] in
self?.viewCamera.refresh()
}
}
//MARK: private
private func confirmTrash(item:MCameraRecord)
{
MSession.sharedInstance.camera?.trashRecord(record:item)
viewCamera.refresh()
}
//MARK: public
func help()
{
let helpCamera:MHelpCamera = MHelpCamera()
let controllerHelp:CHelp = CHelp(model:helpCamera)
parentController.push(
controller:controllerHelp,
vertical:CParent.TransitionVertical.fromTop)
}
func shoot()
{
let controller:CCameraShoot = CCameraShoot()
parentController.push(
controller:controller,
vertical:CParent.TransitionVertical.fromTop)
}
func picker(record:MCameraRecord?)
{
let controller:CCameraPicker = CCameraPicker(camera:self, record:record)
present(controller, animated:true, completion:nil)
}
func trash(item:MCameraRecord)
{
let alert:UIAlertController = UIAlertController(
title:
NSLocalizedString("CCamera_alertTitle", comment:""),
message:nil,
preferredStyle:UIAlertControllerStyle.actionSheet)
let actionCancel:UIAlertAction = UIAlertAction(
title:
NSLocalizedString("CCamera_alertCancel", comment:""),
style:
UIAlertActionStyle.cancel)
let actionDelete:UIAlertAction = UIAlertAction(
title:
NSLocalizedString("CCamera_alertDelete", comment:""),
style:
UIAlertActionStyle.destructive)
{ [weak self] (action:UIAlertAction) in
self?.confirmTrash(item:item)
}
alert.addAction(actionDelete)
alert.addAction(actionCancel)
if let popover:UIPopoverPresentationController = alert.popoverPresentationController
{
popover.sourceView = viewCamera
popover.sourceRect = CGRect.zero
popover.permittedArrowDirections = UIPopoverArrowDirection.up
}
present(alert, animated:true, completion:nil)
}
func rotate(item:MCameraRecord)
{
let controllerRotate:CCameraRotate = CCameraRotate(record:item)
parentController.push(
controller:controllerRotate,
vertical:CParent.TransitionVertical.fromTop)
}
func scale(item:MCameraRecord)
{
let controllerScale:CCameraScale = CCameraScale(record:item)
parentController.push(
controller:controllerScale,
vertical:CParent.TransitionVertical.fromTop)
}
func crop(item:MCameraRecord)
{
let controllerCrop:CCameraCrop = CCameraCrop(record:item)
parentController.push(
controller:controllerCrop,
vertical:CParent.TransitionVertical.fromTop)
}
func showMore(item:MCameraRecord)
{
let controllerMore:CCameraMore = CCameraMore(controller:self, record:item)
parentController.animateOver(controller:controllerMore)
}
func next()
{
MSession.sharedInstance.camera?.buildActiveRecords()
let controller:CCameraFilter = CCameraFilter()
parentController.push(
controller:controller,
horizontal:CParent.TransitionHorizontal.fromRight)
}
func goPlus()
{
let controllerGoPlus:CStoreGoPlus = CStoreGoPlus()
parentController.animateOver(controller:controllerGoPlus)
}
}
| mit | 7231d314a177f99d35b91701f5e894b2 | 25.486607 | 92 | 0.583853 | 5.383848 | false | false | false | false |
WestlakeAPC/game-off-2016 | external/Fiber2D/Fiber2D/LayoutBox.swift | 1 | 3889 | import SwiftMath
/**
Declares the possible directions for laying out nodes in a LayoutBox.
*/
enum LayoutBoxDirection { /// The children will be horizontally aligned.
case horizontal
/// The children will be vertically aligned.
case vertical
}
func roundUpToEven(_ f: Float) -> Float {
return ceilf(f / 2.0) * 2.0
}
/**
The box layout lays out its child nodes in a horizontal row or a vertical column. Optionally you can set a spacing between the child nodes.
@note In order to layout nodes in a grid, you can add one or more LayoutBox as child node with the opposite layout direction, ie the parent
box layout node uses vertical and the child box layout nodes use horizontal LayoutBoxDirection to create a grid of nodes.
*/
class LayoutBox: Layout {
/** @name Layout Options */
/**
The direction is either horizontal or vertical.
@see CCLayoutBoxDirection
*/
var direction: LayoutBoxDirection = .horizontal {
didSet {
needsLayout()
}
}
/**
The spacing in points between the child nodes.
*/
var spacing: Float = 0.0 {
didSet {
needsLayout()
}
}
override func layout() {
super.layout()
guard children.count > 0 else {
return
}
if direction == .horizontal {
// Get the maximum height
var maxHeight: Float = 0
for child in self.children {
let height = Float(child.contentSizeInPoints.height)
if height > maxHeight {
maxHeight = height
}
}
// Position the nodes
var width: Float = 0
for child in self.children {
let childSize: Size = child.contentSizeInPoints
let offset: Point = child.anchorPointInPoints
let localPos: Point = p2d(roundf(width), roundf(maxHeight - childSize.height / 2.0))
let position: Point = localPos + offset
child.position = position
child.positionType = PositionType.points
width += Float(childSize.width)
width += spacing
}
// Account for last added increment
width -= spacing
if width < 0 {
width = 0
}
self.contentSizeType = SizeType.points
self.contentSize = Size(width: Float(roundUpToEven(width)), height: Float(roundUpToEven(maxHeight)))
}
else {
// Get the maximum width
var maxWidth: Float = 0
for child in self.children {
let width = Float(child.contentSizeInPoints.width)
if width > maxWidth {
maxWidth = width
}
}
// Position the nodes
var height: Float = 0
for child in self.children {
let childSize: Size = child.contentSizeInPoints
let offset: Point = child.anchorPointInPoints
let localPos: Point = p2d(Float(roundf((maxWidth - Float(childSize.width)) / 2.0)), Float(roundf(height)))
let position: Point = localPos + offset
child.position = position
child.positionType = PositionType.points
height += Float(childSize.height)
height += spacing
}
// Account for last added increment
height -= spacing
if height < 0 {
height = 0
}
self.contentSizeType = SizeType.points
self.contentSize = Size(width: Float(roundUpToEven(maxWidth)), height: Float(roundUpToEven(height)))
}
}
override func childWasRemoved(child: Node) {
needsLayout()
}
}
| apache-2.0 | ebb90d63b1d6481af0a71a05883753ad | 34.036036 | 140 | 0.555927 | 5.050649 | false | false | false | false |
QuarkWorks/RealmModelGenerator | RealmModelGenerator/Model.swift | 1 | 2278 | //
// Model.swift
// RealmModelGenerator
//
// Created by Brandon Erbschloe on 3/2/16.
// Copyright © 2016 QuarkWorks. All rights reserved.
//
import Foundation
class Model {
static let TAG = NSStringFromClass(Model.self)
weak var schema:Schema!
private(set) var version:String
internal(set) var isModifiable:Bool = true
internal(set) var entities:[Entity] = []
var entitiesByName:[String:Entity] {
get {
var entitiesByName = [String:Entity](minimumCapacity:self.entities.count)
for entity in self.entities {
entitiesByName[entity.name] = entity
}
return entitiesByName
}
}
let observable:Observable
internal init(version:String, schema: Schema) {
self.version = version
self.schema = schema
self.observable = DeferredObservable(observable: schema.observable)
}
func createEntity() -> Entity {
return createEntity(build: {_ in})
}
func createEntity( build: (Entity) throws -> Void) rethrows -> Entity {
var name = "Entity"
var count = 0;
while entities.contains(where: {$0.name == name}) {
count += 1
name = "Entity\(count)"
}
let entity = Entity(name:name, model:self)
try build(entity) //the entity is added to self.entities after it build successfully
entities.append(entity)
self.observable.notifyObservers()
return entity
}
func removeEntity(entity:Entity) {
entity.model = nil
entities.forEach{ (e) in
e.relationships.forEach({ (r) in
if r.destination === entity {
r.destination = nil
}
})
if e.superEntity === entity {
e.superEntity = nil
}
}
if let index = entities.index(where: {$0 === entity}) {
entities.remove(at: index)
}
self.observable.notifyObservers()
}
func setVersion(version:String) {
self.version = version;
}
func isDeleted() -> Bool {
return self.schema == nil
}
}
| mit | 35d9ae93b06654446e2c50fee6810ccb | 25.788235 | 92 | 0.547211 | 4.628049 | false | false | false | false |
digoreis/swift-proposal-analyzer | Sources/Version.swift | 1 | 1971 | //
// Created by Jesse Squires
// http://www.jessesquires.com
//
//
// GitHub
// https://github.com/jessesquires/swift-proposal-analyzer
//
//
// License
// Copyright © 2016 Jesse Squires
// Released under an MIT license: http://opensource.org/licenses/MIT
//
import Foundation
/// Represents the Swift language version.
public struct SwiftVersion {
public let major: Int
public let minor: Int
public let patch: Int
public static let v2_2 = SwiftVersion(major: 2, minor: 2, patch: 0)
public static let v2_3 = SwiftVersion(major: 2, minor: 3, patch: 0)
public static let v3_0 = SwiftVersion(major: 3, minor: 0, patch: 0)
public static let v3_0_1 = SwiftVersion(major: 3, minor: 0, patch: 1)
public static let v3_1 = SwiftVersion(major: 3, minor: 1, patch: 0)
public static let v4_0 = SwiftVersion(major: 4, minor: 0, patch: 0)
}
extension SwiftVersion {
public static let all: [SwiftVersion] = [.v2_2, .v2_3,
.v3_0, .v3_0_1, .v3_1,
.v4_0]
}
extension SwiftVersion: CustomStringConvertible {
public var description: String {
return "\(major).\(minor)" + ((patch != 0) ? ".\(patch)" : "")
}
}
extension SwiftVersion: Equatable {
public static func ==(lhs: SwiftVersion, rhs: SwiftVersion) -> Bool {
return lhs.major == rhs.major
&& lhs.minor == rhs.minor
&& lhs.patch == rhs.patch
}
}
extension SwiftVersion: Comparable {
public static func < (lhs: SwiftVersion, rhs: SwiftVersion) -> Bool {
if lhs.major == rhs.major {
if lhs.minor == rhs.minor {
return lhs.patch < rhs.patch
}
return lhs.minor < rhs.minor
}
return lhs.major < rhs.major
}
}
extension SwiftVersion: Hashable {
public var hashValue: Int {
return major.hashValue ^ minor.hashValue ^ patch.hashValue
}
}
| mit | 2ede88178c756a919474c14554361fe3 | 27.550725 | 73 | 0.596954 | 3.69606 | false | false | false | false |
tardieu/swift | stdlib/private/SwiftPrivate/PRNG.swift | 23 | 1721 | //===--- PRNG.swift -------------------------------------------------------===//
//
// This source file is part of the Swift.org open source project
//
// Copyright (c) 2014 - 2017 Apple Inc. and the Swift project authors
// Licensed under Apache License v2.0 with Runtime Library Exception
//
// See https://swift.org/LICENSE.txt for license information
// See https://swift.org/CONTRIBUTORS.txt for the list of Swift project authors
//
//===----------------------------------------------------------------------===//
import SwiftShims
public func rand32() -> UInt32 {
return _swift_stdlib_cxx11_mt19937()
}
public func rand32(exclusiveUpperBound limit: UInt32) -> UInt32 {
return _swift_stdlib_cxx11_mt19937_uniform(limit)
}
public func rand64() -> UInt64 {
return
(UInt64(_swift_stdlib_cxx11_mt19937()) << 32) |
UInt64(_swift_stdlib_cxx11_mt19937())
}
public func randInt() -> Int {
#if arch(i386) || arch(arm)
return Int(Int32(bitPattern: rand32()))
#elseif arch(x86_64) || arch(arm64) || arch(powerpc64) || arch(powerpc64le) || arch(s390x)
return Int(Int64(bitPattern: rand64()))
#else
fatalError("unimplemented")
#endif
}
public func randArray64(_ count: Int) -> [UInt64] {
var result = [UInt64](repeating: 0, count: count)
for i in result.indices {
result[i] = rand64()
}
return result
}
public func randArray(_ count: Int) -> [Int] {
var result = [Int](repeating: 0, count: count)
for i in result.indices {
result[i] = randInt()
}
return result
}
public func pickRandom<
C : RandomAccessCollection
>(_ c: C) -> C.Iterator.Element {
let i = Int(rand32(exclusiveUpperBound: numericCast(c.count)))
return c[c.index(c.startIndex, offsetBy: numericCast(i))]
}
| apache-2.0 | e3051d36d522e8494338aeb888e4cd9d | 27.683333 | 90 | 0.632191 | 3.541152 | false | false | false | false |
janbiasi/ios-practice | FlickrSearch/FlickrSearch/FlickrPhotosViewController.swift | 1 | 4134 | //
// FlickrPhotosViewController.swift
// FlickrSearch
//
// Created by Jan Biasi on 09.07.15.
// Copyright (c) 2015 Namics AG. All rights reserved.
//
import UIKit
class FlickrPhotosViewController: UICollectionViewController, UICollectionViewDataSource, UITextFieldDelegate, UICollectionViewDelegateFlowLayout {
//MARK: - Private Stuff
private let cellReuseIdentifier = "FlickrCell"
private let collectionReuseIdentifier = "FlickrReusableCollectionView"
private let sectionInsets = UIEdgeInsets(top: 50.0, left: 20.0, bottom: 50.0, right: 20.0)
private var searches = [FlickrSearchResults]()
private let flickr = Flickr()
func photoForIndexPath(indexPath: NSIndexPath) -> FlickrPhoto {
return searches[indexPath.section].searchResults[indexPath.row]
}
//MARK: - UICollectionViewDataSource
override func numberOfSectionsInCollectionView(collectionView: UICollectionView) -> Int {
return searches.count
}
override func collectionView(collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int {
return searches[section].searchResults.count
}
override func collectionView(collectionView: UICollectionView, cellForItemAtIndexPath indexPath: NSIndexPath) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCellWithReuseIdentifier(cellReuseIdentifier, forIndexPath: indexPath) as! FlickrPhotoCell
let flickrPhoto = photoForIndexPath(indexPath)
cell.imageView.image = flickrPhoto.thumbnail
return cell
}
override func collectionView(collectionView: UICollectionView,
viewForSupplementaryElementOfKind kind: String,
atIndexPath indexPath: NSIndexPath) -> UICollectionReusableView {
switch kind {
case UICollectionElementKindSectionHeader:
let headerView =
collectionView.dequeueReusableSupplementaryViewOfKind(kind,
withReuseIdentifier: self.collectionReuseIdentifier,
forIndexPath: indexPath)
as! FlickrPhotoHeaderView
headerView.label.text = searches[indexPath.section].searchTerm
return headerView
default:
assert(false, "Unexpected element kind")
break
}
}
//MARK: - UITextFieldDelegate
func textFieldShouldReturn(textField: UITextField) -> Bool {
let activityIndicator = UIActivityIndicatorView(activityIndicatorStyle: .Gray)
textField.addSubview(activityIndicator)
activityIndicator.frame = textField.bounds
activityIndicator.startAnimating()
flickr.searchFlickrForTerm(textField.text) {
results, error in activityIndicator.removeFromSuperview()
if error != nil {
println("Error searching : \(error)")
}
if results != nil {
println("Found \(results!.searchResults.count) images matching query \(results!.searchTerm)")
self.searches.insert(results!, atIndex: 0)
self.collectionView?.reloadData()
}
}
textField.text = nil
textField.resignFirstResponder()
return true
}
//MARK: - UICollectionViewDelegateFlowLayout
func collectionView(collectionView: UICollectionView,
layout collectionViewLayout: UICollectionViewLayout,
sizeForItemAtIndexPath indexPath: NSIndexPath) -> CGSize {
let flickrPhoto = photoForIndexPath(indexPath)
if var size = flickrPhoto.thumbnail?.size {
size.width += 10
size.height += 10
return size
}
return CGSize(width: 100, height: 100)
}
func collectionView(collectionView: UICollectionView,
layout collectionViewLayout: UICollectionViewLayout,
insetForSectionAtIndex section: Int) -> UIEdgeInsets {
return sectionInsets
}
}
| gpl-2.0 | ecafee3522f770d2bb8778a3818c5e46 | 37.277778 | 147 | 0.65433 | 6.459375 | false | false | false | false |
zhou9734/Warm | Warm/Classes/Me/Controller/MeViewController.swift | 1 | 3915 | //
// MeViewController.swift
// Warm
//
// Created by zhoucj on 16/9/12.
// Copyright © 2016年 zhoucj. All rights reserved.
//
import UIKit
let MeTableCellReuseIdentifier = "MeTableCellReuseIdentifier"
let SettingClickNotication = "settingClickNotication"
class MeViewController: UIViewController {
let meViewModel = MeViewModel()
var wmenus = [WMenu]()
override func viewDidLoad() {
super.viewDidLoad()
setupUI()
NSNotificationCenter.defaultCenter().addObserver(self, selector: Selector("openSetting"), name: SettingClickNotication, object: nil)
}
override func viewWillAppear(animated: Bool) {
super.viewWillAppear(animated)
//隐藏navigationBar
navigationController?.navigationBarHidden = true
tableHeadView.token = Token.loadAccount()
}
private func setupUI(){
wmenus = meViewModel.loadData()
tableFooterView.menu = wmenus.last!.menus!.first
view.addSubview(tableView)
tableView.snp_makeConstraints { (make) -> Void in
make.top.equalTo(view.snp_top).offset(-20)
make.left.right.bottom.equalTo(view)
}
}
private lazy var tableView: UITableView = {
let tv = UITableView(frame: CGRectZero,style: .Plain)
tv.registerClass(UITableViewCell.self, forCellReuseIdentifier: MeTableCellReuseIdentifier)
tv.dataSource = self
tv.delegate = self
tv.backgroundColor = UIColor.whiteColor()
tv.tableHeaderView = self.tableHeadView
tv.tableFooterView = self.tableFooterView
return tv
}()
private lazy var tableHeadView: MeTableHeadView = MeTableHeadView(frame: CGRect(x: 0, y: 0, width: ScreenWidth, height: ScreenHeight*0.3))
private lazy var tableFooterView: MeTableFooterView = MeTableFooterView(frame: CGRect(x: 0, y: 0, width: ScreenWidth, height: 76))
@objc private func openSetting(){
navigationController?.pushViewController(SettingViewController(), animated: true)
}
private func detail(index: Int){
let detailVC = DetailViewController()
if let menu = wmenus[0].menus?[index] {
detailVC.titleName = menu.name
}
navigationController?.pushViewController(detailVC, animated: true)
}
deinit{
NSNotificationCenter.defaultCenter().removeObserver(self)
}
}
//MARK: - UITableViewDataSource代理
extension MeViewController: UITableViewDataSource{
func numberOfSectionsInTableView(tableView: UITableView) -> Int {
return 1
}
func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return wmenus[0].menus?.count ?? 0
}
func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCellWithIdentifier(MeTableCellReuseIdentifier, forIndexPath: indexPath)
if let menu = wmenus[0].menus?[indexPath.row] {
cell.imageView?.image = UIImage(named: menu.image!)
cell.textLabel?.text = menu.name
}
cell.imageView?.frame.size = CGSize(width: 24.0, height: 24.0)
cell.textLabel?.font = UIFont.systemFontOfSize(15)
cell.textLabel?.textColor = UIColor.lightGrayColor()
//设置cell后面箭头样式
cell.accessoryType = .DisclosureIndicator;
//设置选中cell样式
cell.selectionStyle = .Gray;
return cell
}
func tableView(tableView: UITableView, heightForRowAtIndexPath indexPath: NSIndexPath) -> CGFloat {
return 55
}
}
//MARK: - UITableViewDelegate代理
extension MeViewController: UITableViewDelegate{
func tableView(tableView: UITableView, didSelectRowAtIndexPath indexPath: NSIndexPath){
//释放选中效果
tableView.deselectRowAtIndexPath(indexPath, animated: true)
detail(indexPath.row)
}
}
| mit | 3427f4ab4ba1bef099c1997cf1c0b08d | 37.217822 | 142 | 0.688083 | 4.898477 | false | false | false | false |
lieonCX/Live | Live/Model/User/UserInfo.swift | 1 | 1497 | //
// UserInfo.swift
// Live
//
// Created by lieon on 2017/6/20.
// Copyright © 2017年 ChengDuHuanLeHui. All rights reserved.
//
import Foundation
import ObjectMapper
class RegisterInfo: Model {
var status: RegiseterStatus = .success
override func mapping(map: Map) {
status <- map["status"]
}
}
class UserInfo: NSObject, Mappable, NSCoding {
var avatar: String?
var userId: String?
var name: String?
var phone: String?
var uuid: String?
var gender: Gender = .unknown
var coverURL: String?
required public init?(map: Map) {
}
required init?(coder aDecoder: NSCoder) {
self.avatar = aDecoder.decodeObject(forKey: "avastar") as? String
self.userId = aDecoder.decodeObject(forKey: "id") as? String
self.name = aDecoder.decodeObject(forKey: "nickName") as? String
self.phone = aDecoder.decodeObject(forKey: "phone") as? String
self.uuid = aDecoder.decodeObject(forKey: "uuid") as? String
}
func encode(with aCoder: NSCoder) {
aCoder.encode(self.avatar, forKey: "avastar")
aCoder.encode(self.userId, forKey: "id")
aCoder.encode(self.name, forKey: "nickName")
aCoder.encode(self.phone, forKey: "phone")
aCoder.encode(self.uuid, forKey: "uuid")
}
func mapping(map: Map) {
avatar <- map["avastar"]
userId <- map["id"]
name <- map["nickName"]
phone <- map["phone"]
}
}
| mit | 2b33e0836bb7734d6f7ca4dfca8683ad | 26.666667 | 73 | 0.613788 | 3.931579 | false | false | false | false |
HongliYu/firefox-ios | Sync/BatchingClient.swift | 1 | 10289 | /* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/. */
import Foundation
import Alamofire
import Shared
import XCGLogger
import Deferred
open class SerializeRecordFailure<T: CleartextPayloadJSON>: MaybeErrorType, SyncPingFailureFormattable {
public let record: Record<T>
open var failureReasonName: SyncPingFailureReasonName {
return .otherError
}
open var description: String {
return "Failed to serialize record: \(record)"
}
public init(record: Record<T>) {
self.record = record
}
}
private let log = Logger.syncLogger
private typealias UploadRecord = (guid: GUID, payload: String, sizeBytes: Int)
public typealias DeferredResponse = Deferred<Maybe<StorageResponse<POSTResult>>>
typealias BatchUploadFunction = (_ lines: [String], _ ifUnmodifiedSince: Timestamp?, _ queryParams: [URLQueryItem]?) -> Deferred<Maybe<StorageResponse<POSTResult>>>
private let commitParam = URLQueryItem(name: "commit", value: "true")
private enum AccumulateRecordError: MaybeErrorType {
var description: String {
switch self {
case .full:
return "Batch or payload is full."
case .unknown:
return "Unknown errored while trying to accumulate records in batch"
}
}
case full(uploadOp: DeferredResponse)
case unknown
}
open class TooManyRecordsError: MaybeErrorType, SyncPingFailureFormattable {
open var description: String {
return "Trying to send too many records in a single batch."
}
open var failureReasonName: SyncPingFailureReasonName {
return .otherError
}
}
open class RecordsFailedToUpload: MaybeErrorType, SyncPingFailureFormattable {
open var description: String {
return "Some records failed to upload"
}
open var failureReasonName: SyncPingFailureReasonName {
return .otherError
}
}
open class Sync15BatchClient<T: CleartextPayloadJSON> {
fileprivate(set) var ifUnmodifiedSince: Timestamp?
fileprivate let config: InfoConfiguration
fileprivate let uploader: BatchUploadFunction
fileprivate let serializeRecord: (Record<T>) -> String?
fileprivate var batchToken: BatchToken?
// Keep track of the limits of a single batch
fileprivate var totalBytes: ByteCount = 0
fileprivate var totalRecords: Int = 0
// Keep track of the limits of a single POST
fileprivate var postBytes: ByteCount = 0
fileprivate var postRecords: Int = 0
fileprivate var records = [UploadRecord]()
fileprivate var onCollectionUploaded: (POSTResult, Timestamp?) -> DeferredTimestamp
fileprivate func batchQueryParamWithValue(_ value: String) -> URLQueryItem {
return URLQueryItem(name: "batch", value: value)
}
init(config: InfoConfiguration, ifUnmodifiedSince: Timestamp? = nil, serializeRecord: @escaping (Record<T>) -> String?,
uploader: @escaping BatchUploadFunction, onCollectionUploaded: @escaping (POSTResult, Timestamp?) -> DeferredTimestamp) {
self.config = config
self.ifUnmodifiedSince = ifUnmodifiedSince
self.uploader = uploader
self.serializeRecord = serializeRecord
self.onCollectionUploaded = onCollectionUploaded
}
open func endBatch() -> Success {
guard !records.isEmpty else {
return succeed()
}
if let token = self.batchToken {
return commitBatch(token) >>> succeed
}
let lines = self.freezePost()
return self.uploader(lines, self.ifUnmodifiedSince, nil)
>>== effect(moveForward)
>>> succeed
}
// If in batch mode, will discard the batch if any record fails
open func endSingleBatch() -> Deferred<Maybe<(succeeded: [GUID], lastModified: Timestamp?)>> {
return self.start() >>== { response in
let succeeded = response.value.success
guard let token = self.batchToken else {
return deferMaybe((succeeded: succeeded, lastModified: response.metadata.lastModifiedMilliseconds))
}
guard succeeded.count == self.totalRecords else {
return deferMaybe(RecordsFailedToUpload())
}
return self.commitBatch(token) >>== { commitResp in
return deferMaybe((succeeded: succeeded, lastModified: commitResp.metadata.lastModifiedMilliseconds))
}
}
}
open func addRecords(_ records: [Record<T>], singleBatch: Bool = false) -> Success {
guard !records.isEmpty else {
return succeed()
}
// Eagerly serializer the record prior to processing them so we can catch any issues
// with record sizes before we start uploading to the server.
let serializeThunks = records.map { record in
return { self.serialize(record) }
}
return accumulate(serializeThunks) >>== {
let iter = $0.makeIterator()
if singleBatch {
return self.addRecordsInSingleBatch(iter)
} else {
return self.addRecords(iter)
}
}
}
fileprivate func addRecords(_ generator: IndexingIterator<[UploadRecord]>) -> Success {
var mutGenerator = generator
while let record = mutGenerator.next() {
return accumulateOrUpload(record) >>> { self.addRecords(mutGenerator) }
}
return succeed()
}
fileprivate func addRecordsInSingleBatch(_ generator: IndexingIterator<[UploadRecord]>) -> Success {
var mutGenerator = generator
while let record = mutGenerator.next() {
guard self.addToPost(record) else {
return deferMaybe(TooManyRecordsError())
}
}
return succeed()
}
fileprivate func accumulateOrUpload(_ record: UploadRecord) -> Success {
return accumulateRecord(record).bind { result in
// Try to add the record to our buffer
guard let e = result.failureValue as? AccumulateRecordError else {
return succeed()
}
switch e {
case .full(let uploadOp):
return uploadOp >>> { self.accumulateOrUpload(record) }
default:
return deferMaybe(e)
}
}
}
fileprivate func accumulateRecord(_ record: UploadRecord) -> Success {
guard let token = self.batchToken else {
guard addToPost(record) else {
return deferMaybe(AccumulateRecordError.full(uploadOp: self.start()))
}
return succeed()
}
guard fitsInBatch(record) else {
return deferMaybe(AccumulateRecordError.full(uploadOp: self.commitBatch(token)))
}
guard addToPost(record) else {
return deferMaybe(AccumulateRecordError.full(uploadOp: self.postInBatch(token)))
}
addToBatch(record)
return succeed()
}
fileprivate func serialize(_ record: Record<T>) -> Deferred<Maybe<UploadRecord>> {
guard let line = self.serializeRecord(record) else {
return deferMaybe(SerializeRecordFailure(record: record))
}
let lineSize = line.utf8.count
guard lineSize < Sync15StorageClient.maxRecordSizeBytes else {
return deferMaybe(RecordTooLargeError(size: lineSize, guid: record.id))
}
return deferMaybe((record.id, line, lineSize))
}
fileprivate func addToPost(_ record: UploadRecord) -> Bool {
guard postRecords + 1 <= config.maxPostRecords && postBytes + record.sizeBytes <= config.maxPostBytes else {
return false
}
postRecords += 1
postBytes += record.sizeBytes
records.append(record)
return true
}
fileprivate func fitsInBatch(_ record: UploadRecord) -> Bool {
return totalRecords + 1 <= config.maxTotalRecords && totalBytes + record.sizeBytes <= config.maxTotalBytes
}
fileprivate func addToBatch(_ record: UploadRecord) {
totalRecords += 1
totalBytes += record.sizeBytes
}
fileprivate func postInBatch(_ token: BatchToken) -> DeferredResponse {
// Push up the current payload to the server and reset
let lines = self.freezePost()
return uploader(lines, self.ifUnmodifiedSince, [batchQueryParamWithValue(token)])
}
fileprivate func commitBatch(_ token: BatchToken) -> DeferredResponse {
resetBatch()
let lines = self.freezePost()
let queryParams = [batchQueryParamWithValue(token), commitParam]
return uploader(lines, self.ifUnmodifiedSince, queryParams)
>>== effect(moveForward)
}
fileprivate func start() -> DeferredResponse {
let postRecordCount = self.postRecords
let postBytesCount = self.postBytes
let lines = freezePost()
return self.uploader(lines, self.ifUnmodifiedSince, [batchQueryParamWithValue("true")])
>>== effect(moveForward)
>>== { response in
if let token = response.value.batchToken {
self.batchToken = token
// Now that we've started a batch, make sure to set the counters for the batch to include
// the records we just sent as part of the start call.
self.totalRecords = postRecordCount
self.totalBytes = postBytesCount
}
return deferMaybe(response)
}
}
fileprivate func moveForward(_ response: StorageResponse<POSTResult>) {
let lastModified = response.metadata.lastModifiedMilliseconds
self.ifUnmodifiedSince = lastModified
_ = self.onCollectionUploaded(response.value, lastModified)
}
fileprivate func resetBatch() {
totalBytes = 0
totalRecords = 0
self.batchToken = nil
}
fileprivate func freezePost() -> [String] {
let lines = records.map { $0.payload }
self.records = []
self.postBytes = 0
self.postRecords = 0
return lines
}
}
| mpl-2.0 | 60ffc2b6e490b4df2c7ec69036facf8d | 33.996599 | 164 | 0.640393 | 5.02638 | false | false | false | false |
sunshineclt/NKU-Helper | NKU Helper/Structures/ErrorHandler.swift | 1 | 3877 | //
// ErrorHandler.swift
// NKU Helper
//
// Created by 陈乐天 on 15/9/23.
// Copyright © 2015年 陈乐天. All rights reserved.
//
import UIKit
protocol ErrorHandlerProtocol {
static var title:String {get}
static var message:String {get}
static var cancelButtonTitle:String {get}
}
struct ErrorHandler {
struct NetworkError:ErrorHandlerProtocol {
static let title = "网络错误"
static let message = "请检查网络"
static let cancelButtonTitle = "知道啦,我去检查一下!"
}
struct UserNameOrPasswordWrong:ErrorHandlerProtocol {
static let title = "登录失败"
static let message = "用户不存在或密码错误"
static let cancelButtonTitle = "好,重新设置用户名和密码"
}
struct ValidateCodeWrong:ErrorHandlerProtocol {
static let title = "登录失败"
static let message = "验证码错误"
static let cancelButtonTitle = "好,重新输入验证码"
}
struct NotLoggedIn:ErrorHandlerProtocol {
static let title = "尚未登录"
static let message = "登陆后才可使用此功能,请到设置中登陆"
static let cancelButtonTitle = "知道了!"
}
struct CoursesNotExist:ErrorHandlerProtocol {
static let title = "尚未获取课程表"
static let message = "请到课程表页面获取课程表"
static let cancelButtonTitle = "好!"
}
struct GetNotiFailed:ErrorHandlerProtocol {
static let title = "获取通知列表失败"
static let message = "请稍后再试或通知开发者"
static let cancelButtonTitle = "好"
}
struct SelectCourseFail:ErrorHandlerProtocol {
static let title = "选课失败"
static let message = "选课失败"
static let cancelButtonTitle = "好"
}
struct HtmlAnalyseFail:ErrorHandlerProtocol {
static let title = "解析失败"
static let message = "请重试,若仍然出现问题请通知开发者"
static let cancelButtonTitle = "好"
}
struct EvaluateSystemNotOpen:ErrorHandlerProtocol {
static let title = "评教系统未开"
static let message = "若评教系统实际已开放请联系开发者"
static let cancelButtonTitle = "好"
}
struct EvaluateHasDone:ErrorHandlerProtocol {
static let title = "已评教"
static let message = "此课程已评教"
static let cancelButtonTitle = "好"
}
struct EvaluateSubmitFail:ErrorHandlerProtocol {
static let title = "评教失败"
static let message = "评教提交失败"
static let cancelButtonTitle = "好"
}
struct shareFail:ErrorHandlerProtocol {
static let title = "分享失败"
static let message = "请检查网络"
static let cancelButtonTitle = "好"
}
struct DataBaseError:ErrorHandlerProtocol {
static let title = "数据库错误"
static let message = "请重试,若仍然出现问题请通知开发者"
static let cancelButtonTitle = "好"
}
static func alert(withError error:ErrorHandlerProtocol, andHandler handler: ((UIAlertAction) -> Void)? = nil) -> UIAlertController {
let alert = UIAlertController(title: type(of: error).title, message: type(of: error).message, preferredStyle: .alert)
alert.addAction(UIAlertAction(title: type(of: error).cancelButtonTitle, style: .cancel, handler: handler))
return alert
}
static func alertWith(title: String?, message: String?, cancelButtonTitle: String?) -> UIAlertController {
let alert = UIAlertController(title: title, message: message, preferredStyle: .alert)
alert.addAction(UIAlertAction(title: cancelButtonTitle, style: .cancel, handler: nil))
return alert
}
}
| gpl-3.0 | a9be983e10538132f9b67ecdd143ff51 | 33.907216 | 136 | 0.659776 | 4.2325 | false | false | false | false |
ACChe/eidolon | Kiosk/Sale Artwork Details/SaleArtworkDetailsViewController.swift | 1 | 17178 | import UIKit
import ORStackView
import Artsy_UILabels
import Artsy_UIFonts
import ReactiveCocoa
import Artsy_UIButtons
import SDWebImage
class SaleArtworkDetailsViewController: UIViewController {
var allowAnimations = true
var auctionID = AppSetup.sharedState.auctionID
var saleArtwork: SaleArtwork!
var showBuyersPremiumCommand = { () -> RACCommand in
appDelegate().showBuyersPremiumCommand()
}
class func instantiateFromStoryboard(storyboard: UIStoryboard) -> SaleArtworkDetailsViewController {
return storyboard.viewControllerWithID(.SaleArtworkDetail) as! SaleArtworkDetailsViewController
}
lazy var artistInfoSignal: RACSignal = {
let signal = XAppRequest(.Artwork(id: self.saleArtwork.artwork.id)).filterSuccessfulStatusCodes().mapJSON()
return signal.replayLast()
}()
@IBOutlet weak var metadataStackView: ORTagBasedAutoStackView!
@IBOutlet weak var additionalDetailScrollView: ORStackScrollView!
var buyersPremium: () -> (BuyersPremium?) = { appDelegate().sale.buyersPremium }
override func viewDidLoad() {
super.viewDidLoad()
setupMetadataView()
setupAdditionalDetailStackView()
}
override func prepareForSegue(segue: UIStoryboardSegue, sender: AnyObject?) {
if segue == .ZoomIntoArtwork {
let nextViewController = segue.destinationViewController as! SaleArtworkZoomViewController
nextViewController.saleArtwork = saleArtwork
}
}
enum MetadataStackViewTag: Int {
case LotNumberLabel = 1
case ArtistNameLabel
case ArtworkNameLabel
case ArtworkMediumLabel
case ArtworkDimensionsLabel
case ImageRightsLabel
case EstimateTopBorder
case EstimateLabel
case EstimateBottomBorder
case CurrentBidLabel
case CurrentBidValueLabel
case NumberOfBidsPlacedLabel
case BidButton
case BuyersPremium
}
@IBAction func backWasPressed(sender: AnyObject) {
navigationController?.popViewControllerAnimated(true)
}
private func setupMetadataView() {
enum LabelType {
case Serif
case SansSerif
case ItalicsSerif
case Bold
}
func label(type: LabelType, tag: MetadataStackViewTag, fontSize: CGFloat = 16.0) -> UILabel {
let label: UILabel = { () -> UILabel in
switch type {
case .Serif:
return ARSerifLabel()
case .SansSerif:
return ARSansSerifLabel()
case .ItalicsSerif:
return ARItalicsSerifLabel()
case .Bold:
let label = ARSerifLabel()
label.font = UIFont.sansSerifFontWithSize(label.font.pointSize)
return label
}
}()
label.lineBreakMode = .ByWordWrapping
label.font = label.font.fontWithSize(fontSize)
label.tag = tag.rawValue
label.preferredMaxLayoutWidth = 276
return label
}
let hasLotNumber = (saleArtwork.lotNumber != nil)
if let _ = saleArtwork.lotNumber {
let lotNumberLabel = label(.SansSerif, tag: .LotNumberLabel)
lotNumberLabel.font = lotNumberLabel.font.fontWithSize(12)
metadataStackView.addSubview(lotNumberLabel, withTopMargin: "0", sideMargin: "0")
RAC(lotNumberLabel, "text") <~ saleArtwork.viewModel.lotNumberSignal
}
if let artist = artist() {
let artistNameLabel = label(.SansSerif, tag: .ArtistNameLabel)
artistNameLabel.text = artist.name
metadataStackView.addSubview(artistNameLabel, withTopMargin: hasLotNumber ? "10" : "0", sideMargin: "0")
}
let artworkNameLabel = label(.ItalicsSerif, tag: .ArtworkNameLabel)
artworkNameLabel.text = "\(saleArtwork.artwork.title), \(saleArtwork.artwork.date)"
metadataStackView.addSubview(artworkNameLabel, withTopMargin: "10", sideMargin: "0")
if let medium = saleArtwork.artwork.medium {
if medium.isNotEmpty {
let mediumLabel = label(.Serif, tag: .ArtworkMediumLabel)
mediumLabel.text = medium
metadataStackView.addSubview(mediumLabel, withTopMargin: "22", sideMargin: "0")
}
}
if saleArtwork.artwork.dimensions.count > 0 {
let dimensionsLabel = label(.Serif, tag: .ArtworkDimensionsLabel)
dimensionsLabel.text = (saleArtwork.artwork.dimensions as NSArray).componentsJoinedByString("\n")
metadataStackView.addSubview(dimensionsLabel, withTopMargin: "5", sideMargin: "0")
}
retrieveImageRights().filter { (imageRights) -> Bool in
return (imageRights as? String).isNotNilNotEmpty
}.subscribeNext { [weak self] (imageRights) -> Void in
if (imageRights as! String).isNotEmpty {
let rightsLabel = label(.Serif, tag: .ImageRightsLabel)
rightsLabel.text = imageRights as? String
self?.metadataStackView.addSubview(rightsLabel, withTopMargin: "22", sideMargin: "0")
}
}
let estimateTopBorder = UIView()
estimateTopBorder.constrainHeight("1")
estimateTopBorder.tag = MetadataStackViewTag.EstimateTopBorder.rawValue
metadataStackView.addSubview(estimateTopBorder, withTopMargin: "22", sideMargin: "0")
let estimateLabel = label(.Serif, tag: .EstimateLabel)
estimateLabel.text = saleArtwork.viewModel.estimateString
metadataStackView.addSubview(estimateLabel, withTopMargin: "15", sideMargin: "0")
let estimateBottomBorder = UIView()
estimateBottomBorder.constrainHeight("1")
estimateBottomBorder.tag = MetadataStackViewTag.EstimateBottomBorder.rawValue
metadataStackView.addSubview(estimateBottomBorder, withTopMargin: "10", sideMargin: "0")
rac_signalForSelector("viewDidLayoutSubviews").subscribeNext { [weak estimateTopBorder, weak estimateBottomBorder] (_) -> Void in
estimateTopBorder?.drawDottedBorders()
estimateBottomBorder?.drawDottedBorders()
}
let hasBidsSignal = RACObserve(saleArtwork, "highestBidCents").map{ (cents) -> AnyObject! in
return (cents != nil) && ((cents as? NSNumber ?? 0) > 0)
}
let currentBidLabel = label(.Serif, tag: .CurrentBidLabel)
RAC(currentBidLabel, "text") <~ RACSignal.`if`(hasBidsSignal, then: RACSignal.`return`("Current Bid:"), `else`: RACSignal.`return`("Starting Bid:"))
metadataStackView.addSubview(currentBidLabel, withTopMargin: "22", sideMargin: "0")
let currentBidValueLabel = label(.Bold, tag: .CurrentBidValueLabel, fontSize: 27)
RAC(currentBidValueLabel, "text") <~ saleArtwork.viewModel.currentBidSignal()
metadataStackView.addSubview(currentBidValueLabel, withTopMargin: "10", sideMargin: "0")
let numberOfBidsPlacedLabel = label(.Serif, tag: .NumberOfBidsPlacedLabel)
RAC(numberOfBidsPlacedLabel, "text") <~ saleArtwork.viewModel.numberOfBidsWithReserveSignal
metadataStackView.addSubview(numberOfBidsPlacedLabel, withTopMargin: "10", sideMargin: "0")
let bidButton = ActionButton()
bidButton.rac_signalForControlEvents(.TouchUpInside).subscribeNext { [weak self] (_) -> Void in
if let strongSelf = self {
strongSelf.bid(strongSelf.auctionID, saleArtwork: strongSelf.saleArtwork, allowAnimations: strongSelf.allowAnimations)
}
}
saleArtwork.viewModel.forSaleSignal.subscribeNext { [weak bidButton] (forSale) -> Void in
let forSale = forSale as! Bool
let title = forSale ? "BID" : "SOLD"
bidButton?.setTitle(title, forState: .Normal)
}
RAC(bidButton, "enabled") <~ saleArtwork.viewModel.forSaleSignal
bidButton.tag = MetadataStackViewTag.BidButton.rawValue
metadataStackView.addSubview(bidButton, withTopMargin: "40", sideMargin: "0")
if let _ = buyersPremium() {
let buyersPremiumView = UIView()
buyersPremiumView.tag = MetadataStackViewTag.BuyersPremium.rawValue
let buyersPremiumLabel = ARSerifLabel()
buyersPremiumLabel.font = buyersPremiumLabel.font.fontWithSize(16)
buyersPremiumLabel.text = "This work has a "
buyersPremiumLabel.textColor = .artsyHeavyGrey()
let buyersPremiumButton = ARButton()
let title = "buyers premium"
let attributes: [String: AnyObject] = [ NSUnderlineStyleAttributeName: NSUnderlineStyle.StyleSingle.rawValue, NSFontAttributeName: buyersPremiumLabel.font ];
let attributedTitle = NSAttributedString(string: title, attributes: attributes)
buyersPremiumButton.setTitle(title, forState: .Normal)
buyersPremiumButton.titleLabel?.attributedText = attributedTitle;
buyersPremiumButton.setTitleColor(.artsyHeavyGrey(), forState: .Normal)
buyersPremiumButton.rac_command = showBuyersPremiumCommand()
buyersPremiumView.addSubview(buyersPremiumLabel)
buyersPremiumView.addSubview(buyersPremiumButton)
buyersPremiumLabel.alignTop("0", leading: "0", bottom: "0", trailing: nil, toView: buyersPremiumView)
buyersPremiumLabel.alignBaselineWithView(buyersPremiumButton, predicate: nil)
buyersPremiumButton.alignAttribute(.Left, toAttribute: .Right, ofView: buyersPremiumLabel, predicate: "0")
metadataStackView.addSubview(buyersPremiumView, withTopMargin: "30", sideMargin: "0")
}
metadataStackView.bottomMarginHeight = CGFloat(NSNotFound)
}
private func setupImageView(imageView: UIImageView) {
if let image = saleArtwork.artwork.defaultImage {
// We'll try to retrieve the thumbnail image from the cache. If we don't have it, we'll set the background colour to grey to indicate that we're downloading it.
let key = SDWebImageManager.sharedManager().cacheKeyForURL(image.thumbnailURL())
let thumbnailImage = SDImageCache.sharedImageCache().imageFromDiskCacheForKey(key)
if thumbnailImage == nil {
imageView.backgroundColor = .artsyLightGrey()
}
imageView.sd_setImageWithURL(image.fullsizeURL(), placeholderImage: thumbnailImage, completed: { (image, _, _, _) -> Void in
// If the image was successfully downloaded, make sure we aren't still displaying grey.
if image != nil {
imageView.backgroundColor = .clearColor()
}
})
let heightConstraintNumber = { () -> CGFloat in
if let aspectRatio = image.aspectRatio {
if aspectRatio != 0 {
return min(400, CGFloat(538) / aspectRatio)
}
}
return 400
}()
imageView.constrainHeight( "\(heightConstraintNumber)" )
imageView.contentMode = .ScaleAspectFit
imageView.userInteractionEnabled = true
let recognizer = UITapGestureRecognizer()
imageView.addGestureRecognizer(recognizer)
recognizer.rac_gestureSignal().subscribeNext() { [weak self] (_) in
self?.performSegue(.ZoomIntoArtwork)
return
}
}
}
private func setupAdditionalDetailStackView() {
enum LabelType {
case Header
case Body
}
func label(type: LabelType, layoutSignal: RACSignal? = nil) -> UILabel {
let (label, fontSize) = { () -> (UILabel, CGFloat) in
switch type {
case .Header:
return (ARSansSerifLabel(), 14)
case .Body:
return (ARSerifLabel(), 16)
}
}()
label.font = label.font.fontWithSize(fontSize)
label.lineBreakMode = .ByWordWrapping
layoutSignal?.take(1).subscribeNext { [weak label] (_) -> Void in
if let label = label {
label.preferredMaxLayoutWidth = CGRectGetWidth(label.frame)
}
}
return label
}
additionalDetailScrollView.stackView.bottomMarginHeight = 40
let imageView = UIImageView()
additionalDetailScrollView.stackView.addSubview(imageView, withTopMargin: "0", sideMargin: "40")
setupImageView(imageView)
let additionalInfoHeaderLabel = label(.Header)
additionalInfoHeaderLabel.text = "Additional Information"
additionalDetailScrollView.stackView.addSubview(additionalInfoHeaderLabel, withTopMargin: "20", sideMargin: "40")
if let blurb = saleArtwork.artwork.blurb {
let blurbLabel = label(.Body, layoutSignal: additionalDetailScrollView.stackView.rac_signalForSelector("layoutSubviews"))
blurbLabel.attributedText = MarkdownParser().attributedStringFromMarkdownString( blurb )
additionalDetailScrollView.stackView.addSubview(blurbLabel, withTopMargin: "22", sideMargin: "40")
}
let additionalInfoLabel = label(.Body, layoutSignal: additionalDetailScrollView.stackView.rac_signalForSelector("layoutSubviews"))
additionalInfoLabel.attributedText = MarkdownParser().attributedStringFromMarkdownString( saleArtwork.artwork.additionalInfo )
additionalDetailScrollView.stackView.addSubview(additionalInfoLabel, withTopMargin: "22", sideMargin: "40")
retrieveAdditionalInfo().filter { (info) -> Bool in
return (info as? String).isNotNilNotEmpty
}.subscribeNext { (info) -> Void in
additionalInfoLabel.attributedText = MarkdownParser().attributedStringFromMarkdownString( info as! String )
}
if let artist = artist() {
retrieveArtistBlurb().filter { (blurb) -> Bool in
return (blurb as? String).isNotNilNotEmpty
}.subscribeNext { [weak self] (blurb) -> Void in
if self == nil {
return
}
let aboutArtistHeaderLabel = label(.Header)
aboutArtistHeaderLabel.text = "About \(artist.name)"
self?.additionalDetailScrollView.stackView.addSubview(aboutArtistHeaderLabel, withTopMargin: "22", sideMargin: "40")
let aboutAristLabel = label(.Body, layoutSignal: self?.additionalDetailScrollView.stackView.rac_signalForSelector("layoutSubviews"))
aboutAristLabel.attributedText = MarkdownParser().attributedStringFromMarkdownString( blurb as? String )
self?.additionalDetailScrollView.stackView.addSubview(aboutAristLabel, withTopMargin: "22", sideMargin: "40")
}
}
}
private func artist() -> Artist? {
return saleArtwork.artwork.artists?.first
}
private func retrieveImageRights() -> RACSignal {
let artwork = saleArtwork.artwork
if let imageRights = artwork.imageRights {
return RACSignal.`return`(imageRights)
} else {
return artistInfoSignal.map{ (json) -> AnyObject! in
return json["image_rights"]
}.filter({ (imageRights) -> Bool in
imageRights != nil
}).doNext{ (imageRights) -> Void in
artwork.imageRights = imageRights as? String
return
}
}
}
private func retrieveAdditionalInfo() -> RACSignal {
let artwork = saleArtwork.artwork
if let additionalInfo = artwork.additionalInfo {
return RACSignal.`return`(additionalInfo)
} else {
return artistInfoSignal.map{ (json) -> AnyObject! in
return json["additional_information"]
}.filter({ (info) -> Bool in
info != nil
}).doNext{ (info) -> Void in
artwork.additionalInfo = info as? String
return
}
}
}
private func retrieveArtistBlurb() -> RACSignal {
if let artist = artist() {
if let blurb = artist.blurb {
return RACSignal.`return`(blurb)
} else {
let artistSignal = XAppRequest(.Artist(id: artist.id)).filterSuccessfulStatusCodes().mapJSON()
return artistSignal.map{ (json) -> AnyObject! in
return json["blurb"]
}.filter({ (blurb) -> Bool in
blurb != nil
}).doNext{ (blurb) -> Void in
artist.blurb = blurb as? String
return
}
}
} else {
return RACSignal.empty()
}
}
}
| mit | 38950d5f90a0b88793728783dc821598 | 42.160804 | 172 | 0.627954 | 5.872821 | false | false | false | false |
wireapp/wire-ios-sync-engine | Source/UserSession/ClientUpdateNotification.swift | 1 | 3307 | //
// Wire
// Copyright (C) 2016 Wire Swiss GmbH
//
// This program is free software: you can redistribute it and/or modify
// it under the terms of the GNU General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// This program is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
// You should have received a copy of the GNU General Public License
// along with this program. If not, see http://www.gnu.org/licenses/.
//
import WireDataModel
@objc public enum ZMClientUpdateNotificationType: Int {
case fetchCompleted
case fetchFailed
case deletionCompleted
case deletionFailed
}
@objc public class ZMClientUpdateNotification: NSObject {
private static let name = Notification.Name(rawValue: "ZMClientUpdateNotification")
private static let clientObjectIDsKey = "clientObjectIDs"
private static let typeKey = "notificationType"
private static let errorKey = "error"
@objc public static func addObserver(context: NSManagedObjectContext, block: @escaping (ZMClientUpdateNotificationType, [NSManagedObjectID], NSError?) -> Void) -> Any {
return NotificationInContext.addObserver(name: self.name,
context: context.notificationContext) { note in
guard let type = note.userInfo[self.typeKey] as? ZMClientUpdateNotificationType else { return }
let clientObjectIDs = (note.userInfo[self.clientObjectIDsKey] as? [NSManagedObjectID]) ?? []
let error = note.userInfo[self.errorKey] as? NSError
block(type, clientObjectIDs, error)
}
}
static func notify(type: ZMClientUpdateNotificationType, context: NSManagedObjectContext, clients: [UserClient] = [], error: NSError? = nil) {
NotificationInContext(name: self.name, context: context.notificationContext, userInfo: [
errorKey: error as Any,
clientObjectIDsKey: clients.objectIDs,
typeKey: type
]).post()
}
@objc
public static func notifyFetchingClientsCompleted(userClients: [UserClient], context: NSManagedObjectContext) {
self.notify(type: .fetchCompleted, context: context, clients: userClients)
}
@objc
public static func notifyFetchingClientsDidFail(error: NSError, context: NSManagedObjectContext) {
self.notify(type: .fetchFailed, context: context, error: error)
}
@objc
public static func notifyDeletionCompleted(remainingClients: [UserClient], context: NSManagedObjectContext) {
self.notify(type: .deletionCompleted, context: context, clients: remainingClients)
}
@objc
public static func notifyDeletionFailed(error: NSError, context: NSManagedObjectContext) {
self.notify(type: .deletionFailed, context: context, error: error)
}
}
extension Array where Element: NSManagedObject {
var objectIDs: [NSManagedObjectID] {
return self.compactMap { obj in
guard !obj.objectID.isTemporaryID else { return nil }
return obj.objectID
}
}
}
| gpl-3.0 | 63d330575d5589d8d639e652d69f1335 | 38.843373 | 172 | 0.706078 | 4.731044 | false | false | false | false |
shmidt/StyleItTest | StyleItTest/BoardsVC.swift | 1 | 3879 | //
// BoardsVC.swift
// StyleItTest
//
// Created by Dmitry Shmidt on 7/17/15.
// Copyright (c) 2015 Dmitry Shmidt. All rights reserved.
//
import UIKit
import RealmSwift
import UIKit
import CollectionViewWaterfallLayout
import Alamofire
class BoardsVC: UICollectionViewController, CollectionViewWaterfallLayoutDelegate {
private let reuseIdentifier = "Cell"
var notificationToken: NotificationToken?
var items = Realm().objects(Board)
deinit{
if let notificationToken = notificationToken{
Realm().removeNotification(notificationToken)
}
}
func setupView() {
let layout = CollectionViewWaterfallLayout()
layout.sectionInset = UIEdgeInsets(top: 10, left: 10, bottom: 10, right: 10)
layout.minimumColumnSpacing = 10
layout.minimumInteritemSpacing = 10
collectionView?.collectionViewLayout = layout
collectionView?.alwaysBounceVertical = true
let nibCell = UINib(nibName: "ThumbnailCell", bundle:nil)
self.collectionView?.registerNib(nibCell, forCellWithReuseIdentifier: reuseIdentifier)
}
override func viewDidLoad() {
super.viewDidLoad()
setupView()
// Set realm notification block
notificationToken = Realm().addNotificationBlock { [unowned self] note, realm in
self.collectionView?.reloadData()
}
reloadThumbnails()
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
// MARK: UICollectionViewDelegate
override func collectionView(collectionView: UICollectionView, didSelectItemAtIndexPath indexPath: NSIndexPath) {
let vc = storyboard?.instantiateViewControllerWithIdentifier("StylesVC") as! StylesVC
vc.board = boardAtIndexPath(indexPath)
showViewController(vc, sender: self)
}
override func numberOfSectionsInCollectionView(collectionView: UICollectionView) -> Int {
return 1
}
override func collectionView(collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int {
return items.count
}
override func collectionView(collectionView: UICollectionView, cellForItemAtIndexPath indexPath: NSIndexPath) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCellWithReuseIdentifier(reuseIdentifier, forIndexPath: indexPath) as! ThumbnailCell
let board = boardAtIndexPath(indexPath)
cell.imageView.image = board.thumbnail
cell.request?.cancel()
if board.thumbnail == nil {
println("No thumbnail, downloading it")
// Download from the internet
if let url = board.thumbnailUrl{
cell.downloadImage(url, completion: { (image) -> Void in
let realm = Realm()
realm.write {
board.thumbnail = image
}
})
}
}
return cell
}
// MARK: WaterfallLayoutDelegate
func collectionView(collectionView: UICollectionView, layout: UICollectionViewLayout, sizeForItemAtIndexPath indexPath: NSIndexPath) -> CGSize {
let board = boardAtIndexPath(indexPath)
return board.size
}
//MARK: - Utilities
func reloadThumbnails(){
StyleItManagerSingleton.updateBoardsFromServer { [unowned self](results, error) -> Void in
self.collectionView?.reloadData()
}
}
@IBAction func reloadData(sender: UIBarButtonItem) {
reloadThumbnails()
}
func boardAtIndexPath(indexPath: NSIndexPath) -> Board{
let board = items[indexPath.row]
return board
}
}
| mit | 06624b017cd06129c8f94d7a2c81ea7d | 30.795082 | 148 | 0.647332 | 5.859517 | false | false | false | false |
tzef/BmoViewPager | Example/BmoViewPager/CustomView/UnderLineView.swift | 1 | 1200 | //
// UnderLineView.swift
// BmoViewPager
//
// Created by LEE ZHE YU on 2017/6/4.
// Copyright © 2017年 CocoaPods. All rights reserved.
//
import UIKit
@IBDesignable
class UnderLineView: UIView {
@IBInspectable var strokeColor: UIColor = UIColor.black {
didSet {
self.setNeedsDisplay()
}
}
@IBInspectable var lineWidth: CGFloat = 2.0 {
didSet {
self.setNeedsDisplay()
}
}
@IBInspectable var marginX: CGFloat = 0.0 {
didSet {
self.setNeedsDisplay()
}
}
init() {
super.init(frame: CGRect.zero)
self.backgroundColor = UIColor.clear
}
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
override func draw(_ rect: CGRect) {
super.draw(rect)
guard let context = UIGraphicsGetCurrentContext() else {
return
}
strokeColor.setStroke()
context.setLineWidth(lineWidth)
context.move(to: CGPoint(x: rect.minX + marginX, y: rect.maxY))
context.addLine(to: CGPoint(x: rect.maxX - marginX, y: rect.maxY))
context.strokePath()
}
}
| mit | 9a54e6e7800a0a4f21c95bd24873661c | 25.021739 | 74 | 0.592314 | 4.290323 | false | false | false | false |
waterskier2007/NVActivityIndicatorView | NVActivityIndicatorView/Animations/NVActivityIndicatorAnimationBallZigZagDeflect.swift | 1 | 3778 | //
// NVActivityIndicatorAnimationBallZigZagDeflect.swift
// NVActivityIndicatorViewDemo
//
// The MIT License (MIT)
// Copyright (c) 2016 Vinh Nguyen
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
// The above copyright notice and this permission notice shall be included in all
// copies or substantial portions of the Software.
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
// SOFTWARE.
//
import UIKit
class NVActivityIndicatorAnimationBallZigZagDeflect: NVActivityIndicatorAnimationDelegate {
func setUpAnimation(in layer: CALayer, size: CGSize, color: UIColor) {
let circleSize: CGFloat = size.width / 5
let duration: CFTimeInterval = 0.75
let deltaX = size.width / 2 - circleSize / 2
let deltaY = size.height / 2 - circleSize / 2
<<<<<<< HEAD
let frame = CGRect(x: (layer.bounds.width - circleSize) / 2, y: (layer.bounds.height - circleSize) / 2, width: circleSize, height: circleSize)
=======
let frame = CGRect(x: (layer.bounds.size.width - circleSize) / 2, y: (layer.bounds.size.height - circleSize) / 2, width: circleSize, height: circleSize)
>>>>>>> ninjaprox/master
// Circle 1 animation
let animation = CAKeyframeAnimation(keyPath: "transform")
animation.keyTimes = [0.0, 0.33, 0.66, 1.0]
animation.timingFunction = CAMediaTimingFunction(name: kCAMediaTimingFunctionLinear)
animation.values = [
NSValue(caTransform3D: CATransform3DMakeTranslation(0, 0, 0)),
NSValue(caTransform3D: CATransform3DMakeTranslation(-deltaX, -deltaY, 0)),
NSValue(caTransform3D: CATransform3DMakeTranslation(deltaX, -deltaY, 0)),
NSValue(caTransform3D: CATransform3DMakeTranslation(0, 0, 0)),
]
animation.duration = duration
animation.repeatCount = HUGE
animation.autoreverses = true
animation.isRemovedOnCompletion = false
// Draw circle 1
circleAt(frame: frame, layer: layer, size: CGSize(width: circleSize, height: circleSize), color: color, animation: animation)
// Circle 2 animation
animation.values = [
NSValue(caTransform3D: CATransform3DMakeTranslation(0, 0, 0)),
NSValue(caTransform3D: CATransform3DMakeTranslation(deltaX, deltaY, 0)),
NSValue(caTransform3D: CATransform3DMakeTranslation(-deltaX, deltaY, 0)),
NSValue(caTransform3D: CATransform3DMakeTranslation(0, 0, 0)),
]
// Draw circle 2
circleAt(frame: frame, layer: layer, size: CGSize(width: circleSize, height: circleSize), color: color, animation: animation)
}
func circleAt(frame: CGRect, layer: CALayer, size: CGSize, color: UIColor, animation: CAAnimation) {
let circle = NVActivityIndicatorShape.circle.layerWith(size: size, color: color)
circle.frame = frame
circle.add(animation, forKey: "animation")
layer.addSublayer(circle)
}
}
| mit | af467d4f8f58e6a57a563cd72c83f8a4 | 44.518072 | 160 | 0.699576 | 4.590522 | false | false | false | false |
andreamazz/Tropos | Sources/Tropos/ViewControllers/SettingsTableViewController.swift | 1 | 5463 | import UIKit
import TroposCore
private enum SegueIdentifier: String {
case privacyPolicy = "ShowWebViewController"
case acknowledgements = "ShowTextViewController"
init?(identifier: String?) {
guard let identifier = identifier else { return nil }
self.init(rawValue: identifier)
}
}
enum Section: Int {
case unitSystem
case info
case about
}
enum UnitSystemSection: Int {
case metric
case imperial
}
enum InfoSection: Int {
case privacyPolicy
case acknowledgements
case forecast
}
enum AboutSection: Int {
case thoughtbot
}
class SettingsTableViewController: UITableViewController {
@IBOutlet var thoughtbotImageView: UIImageView!
private var resignActiveObservation: Any?
private let settingsController = SettingsController()
override func viewDidLoad() {
super.viewDidLoad()
settingsController.unitSystemChanged = { [weak self] unitSystem in
guard let indexPath = self?.indexPathForUnitSystem(unitSystem) else { return }
self?.tableView.checkCellAtIndexPath(indexPath)
}
thoughtbotImageView.tintColor = .lightText
thoughtbotImageView.image = thoughtbotImageView.image?.withRenderingMode(
.alwaysTemplate
)
resignActiveObservation = NotificationCenter.default.addObserver(
forName: .UIApplicationWillResignActive,
object: nil,
queue: nil
) { [weak self] _ in
guard let selectedIndexPath = self?.tableView.indexPathForSelectedRow else { return }
self?.tableView.deselectRow(at: selectedIndexPath, animated: true)
}
}
deinit {
if let resignActiveObservation = resignActiveObservation {
NotificationCenter.default.removeObserver(resignActiveObservation)
}
}
override func viewWillAppear(_ animated: Bool) {
super.viewWillAppear(animated)
tableView.checkCellAtIndexPath(indexPathForUnitSystem(settingsController.unitSystem))
}
override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
switch SegueIdentifier(identifier: segue.identifier) {
case .privacyPolicy?:
let webViewController = segue.destination as? WebViewController
webViewController?.url = URL(string: "http://www.troposweather.com/privacy/")!
case .acknowledgements?:
let textViewController = segue.destination as? TextViewController
let fileURL = Bundle.main.url(
forResource: "Pods-Tropos-settings-metadata",
withExtension: "plist"
)
let parser = fileURL.flatMap { AcknowledgementsParser(fileURL: $0) }
textViewController?.text = parser?.displayString()
case nil:
break
}
}
override func tableView(
_ tableView: UITableView,
didSelectRowAt
indexPath: IndexPath
) {
switch (indexPath.section, indexPath.row) {
case (Section.unitSystem.rawValue, _):
tableView.deselectRow(at: indexPath, animated: true)
tableView.uncheckCellsInSection(indexPath.section)
selectUnitSystemAtIndexPath(indexPath)
case (Section.info.rawValue, InfoSection.forecast.rawValue):
UIApplication.shared.openURL(URL(string: "https://forecast.io")!)
case (Section.about.rawValue, AboutSection.thoughtbot.rawValue):
UIApplication.shared.openURL(URL(string: "https://thoughtbot.com")!)
default: break
}
}
override func tableView(
_ tableView: UITableView,
willDisplayHeaderView view: UIView,
forSection section: Int
) {
if let headerView = view as? UITableViewHeaderFooterView {
headerView.textLabel?.font = .defaultLightFont(size: 13)
headerView.textLabel?.textColor = .lightText
}
}
override func tableView(
_ tableView: UITableView,
titleForHeaderInSection section: Int
) -> String? {
switch section {
case Section.about.rawValue: return appVersionString()
default: return super.tableView(tableView, titleForHeaderInSection: section)
}
}
private func appVersionString() -> String? {
let bundle = Bundle.main
guard let infoDictionary = bundle.infoDictionary as? [String: String] else {
return .none
}
guard let version = infoDictionary["CFBundleShortVersionString"] else { return .none }
guard let buildNumber = infoDictionary["CFBundleVersion"] else { return .none }
return "Tropos \(version) (\(buildNumber))".uppercased()
}
private func selectUnitSystemAtIndexPath(_ indexPath: IndexPath) {
if let system = UnitSystem(rawValue: indexPath.row) {
settingsController.unitSystem = system
}
}
private func indexPathForUnitSystem(_ unitSystem: UnitSystem) -> IndexPath {
return IndexPath(row: unitSystem.rawValue, section: 0)
}
}
extension UITableView {
@objc func checkCellAtIndexPath(_ indexPath: IndexPath) {
cellForRow(at: indexPath)?.accessoryType = .checkmark
}
@objc func uncheckCellsInSection(_ section: Int) {
for index in 0 ..< numberOfRows(inSection: section) {
let indexPath = IndexPath(row: index, section: section)
cellForRow(at: indexPath)?.accessoryType = .none
}
}
}
| mit | 9ca7117dd90750131729530fd34c68d5 | 31.909639 | 95 | 0.661724 | 5.197907 | false | false | false | false |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.