repo_name
stringlengths 6
91
| path
stringlengths 8
968
| copies
stringclasses 210
values | size
stringlengths 2
7
| content
stringlengths 61
1.01M
| license
stringclasses 15
values | hash
stringlengths 32
32
| line_mean
float64 6
99.8
| line_max
int64 12
1k
| alpha_frac
float64 0.3
0.91
| ratio
float64 2
9.89
| autogenerated
bool 1
class | config_or_test
bool 2
classes | has_no_keywords
bool 2
classes | has_few_assignments
bool 1
class |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
extremebytes/CodeViews | CodeViews/ViewController.swift | 1 | 8004 | //
// ViewController.swift
// CodeViews
//
// Created by John Woolsey on 2/23/15.
// Copyright (c) 2015 ExtremeBytes Software. All rights reserved.
//
import UIKit
class ViewController: UIViewController {
// MARK: - Properties
/// Dictionary containing all views within the view hierarchy.
var viewsDictionary: Dictionary<String,UIView> = Dictionary()
/// Colored view width; 50 for iPhone 6 Plus and larger sizes, otherwise 0.
var coloredViewWidth: Int {
let screenWidth = Int(UIScreen.mainScreen().bounds.width)
let minimumLabelWidth = 301
let spacingWidth = 8
let colorViewWidth = 50
return screenWidth - minimumLabelWidth - 3 * spacingWidth > colorViewWidth ? colorViewWidth : 0
}
// MARK: - View Lifecycle
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
override func loadView() {
loadTopView()
loadSubViews()
finalizeViews()
}
// MARK: - Custom
/**
Adds the specified view to the top level view hierarchy.
- parameter viewName: The textual name of the view for use in visual formatting.
- parameter viewObject: The actual name of the view object.
*/
func addViewToHierarchy(viewName viewName: String, viewObject: UIView) {
viewObject.translatesAutoresizingMaskIntoConstraints = false
view.addSubview(viewObject)
viewsDictionary[viewName] = viewObject
}
/**
Creates and returns a circle image.
- returns: An optional UIImage of a circle.
*/
func circleImage() -> UIImage? {
let imageSize = CGSize(width: 500, height: 500)
let strokeWidth = CGFloat(20)
let circlePath = UIBezierPath(ovalInRect: CGRect(x: strokeWidth/2, y: strokeWidth/2, width: imageSize.width - strokeWidth, height: imageSize.height - strokeWidth))
UIGraphicsBeginImageContext(CGSize(width: imageSize.width, height: imageSize.height))
circlePath.lineWidth = strokeWidth
UIColor.blackColor().setStroke()
circlePath.stroke()
UIColor.cyanColor().setFill()
circlePath.fill()
let circleImage:UIImage? = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
return circleImage
}
/**
Finalizes the top level view hierarchy.
*/
func finalizeViews() {
// Create buffer view
let bufferView = UIView()
addViewToHierarchy(viewName: "bufferView", viewObject: bufferView)
// Finalize layout constraints
view.addConstraints(NSLayoutConstraint.constraintsWithVisualFormat("V:|-50-[redLabel]-[yellowLabel]-[greenLabel]-[bufferView][circleImageView]-20-|",
options: [], metrics: nil, views: viewsDictionary))
}
/**
Creates and adds the geometric views along with their associated layout constraints to the top level view hierarchy.
*/
func loadGeometricViews() {
// Create circle image view
let circleImageView = UIImageView(image: circleImage())
circleImageView.contentMode = .ScaleAspectFit
addViewToHierarchy(viewName: "circleImageView", viewObject: circleImageView)
// Create square view
let squareView = SquareView()
addViewToHierarchy(viewName: "squareView", viewObject: squareView)
// Create layout constraints for geometric views
view.addConstraints(NSLayoutConstraint.constraintsWithVisualFormat("H:|-[circleImageView]-20-[squareView(==circleImageView)]-|",
options: .AlignAllCenterY, metrics: nil, views: viewsDictionary))
view.addConstraint(NSLayoutConstraint(item: circleImageView, attribute: .Height, relatedBy: .Equal, toItem: circleImageView, attribute: .Width, multiplier: 1, constant: 0))
view.addConstraint(NSLayoutConstraint(item: squareView, attribute: .Height, relatedBy: .Equal, toItem: squareView, attribute: .Width, multiplier: 1, constant: 0))
}
/**
Creates and adds the green views along with their associated layout constraints to the top level view hierarchy.
*/
func loadGreenViews() {
// Create green label
let greenLabel = UILabel()
greenLabel.text = "Green Label"
addViewToHierarchy(viewName: "greenLabel", viewObject: greenLabel)
// Create green view
let greenView = UIView()
greenView.backgroundColor = UIColor.greenColor()
addViewToHierarchy(viewName: "greenView", viewObject: greenView)
// Create layout constraints for green views
view.addConstraints(NSLayoutConstraint.constraintsWithVisualFormat("H:|-[greenView(coloredViewWidth)]-[greenLabel]-|",
options: .AlignAllCenterY, metrics: ["coloredViewWidth":coloredViewWidth], views: viewsDictionary))
view.addConstraints(NSLayoutConstraint.constraintsWithVisualFormat("V:[greenView(==greenLabel)]",
options: [], metrics: nil, views: viewsDictionary))
}
/**
Creates and adds the red views along with their associated layout constraints to the top level view hierarchy.
*/
func loadRedViews() {
// Create red label
let redLabel = UILabel()
redLabel.text = "Red Label"
addViewToHierarchy(viewName: "redLabel", viewObject: redLabel)
// Create red view
let redView = UIView()
redView.backgroundColor = UIColor.redColor()
addViewToHierarchy(viewName: "redView", viewObject: redView)
// Create layout constraints for red views
view.addConstraints(NSLayoutConstraint.constraintsWithVisualFormat("H:|-[redView(coloredViewWidth)]-[redLabel]-|",
options: .AlignAllCenterY, metrics: ["coloredViewWidth":coloredViewWidth], views: viewsDictionary))
view.addConstraints(NSLayoutConstraint.constraintsWithVisualFormat("V:[redView(==redLabel)]",
options: [], metrics: nil, views: viewsDictionary))
}
/**
Creates and adds all subviews along with their associated layout constraints to the top level view hierarchy.
*/
func loadSubViews() {
loadRedViews()
loadYellowViews()
loadGreenViews()
loadGeometricViews()
}
/**
Creates the top level view.
*/
func loadTopView() {
let topView = UIView()
topView.backgroundColor = UIColor.whiteColor()
view = topView
}
/**
Creates and adds the yellow views along with their associated layout constraints to the view hierarchy.
*/
func loadYellowViews() {
// Create yellow label
let yellowLabel = UILabel()
yellowLabel.text = "Yellow Label"
addViewToHierarchy(viewName: "yellowLabel", viewObject: yellowLabel)
// Create yellow view
let yellowView = UIView()
yellowView.backgroundColor = UIColor.yellowColor()
addViewToHierarchy(viewName: "yellowView", viewObject: yellowView)
// Create layout constraints for yellow views
view.addConstraints(NSLayoutConstraint.constraintsWithVisualFormat("H:|-[yellowView(coloredViewWidth)]-[yellowLabel]-|",
options: .AlignAllCenterY, metrics: ["coloredViewWidth":coloredViewWidth], views: viewsDictionary))
view.addConstraints(NSLayoutConstraint.constraintsWithVisualFormat("V:[yellowView(==yellowLabel)]",
options: [], metrics: nil, views: viewsDictionary))
}
/**
Reloads all subviews along with their associated layout constraints in the top level view hierarchy.
*/
func reloadSubviews() {
unloadSubviews()
loadSubViews()
finalizeViews()
}
/**
Removes all subviews and layout constraints from the top level view hierarchy.
*/
func unloadSubviews() {
view.removeConstraints(view.constraints)
for subview in view.subviews {
subview.removeFromSuperview()
}
}
}
| mit | a3e4be6bc3a95ebadce8c9bb713c9490 | 33.951965 | 178 | 0.686907 | 5.379032 | false | false | false | false |
colbylwilliams/bugtrap | iOS/Code/Swift/bugTrap/bugTrapKit/Trackers/Common/TrackerInstance.swift | 1 | 737 | //
// TrackerBase.swift
// bugTrap
//
// Created by Colby L Williams on 11/14/14.
// Copyright (c) 2014 bugTrap. All rights reserved.
//
import Foundation
class TrackerInstance<TState : TrackerState, TProxy : TrackerProxy> : Tracker {
var State : TState?
var Proxy : TProxy?
override init(trackerType: TrackerType) {
super.init(trackerType: trackerType)
_ = keychain.GetStoredKeyValues()
//create the specific state and proxy types
self.State = TState()
//self.Proxy = TProxy(data: trackerData)
self.Proxy = TProxy()
self.trackerState = State
self.trackerProxy = Proxy
TrackerDetails = trackerState.TrackerDetails
}
} | mit | e4017ef4d9d7d957a77a13b3d76c98ec | 22.806452 | 79 | 0.63365 | 4.117318 | false | false | false | false |
mikaoj/BSImagePicker | Sources/Scene/Preview/PreviewViewController.swift | 1 | 8035 | // The MIT License (MIT)
//
// Copyright (c) 2015 Joakim Gyllström
//
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all
// copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
// SOFTWARE.
import UIKit
import Photos
import CoreLocation
class PreviewViewController : UIViewController {
private let imageManager = PHCachingImageManager.default()
var settings: Settings!
var asset: PHAsset? {
didSet {
updateNavigationTitle()
guard let asset = asset else {
imageView.image = nil
return
}
// Load image for preview
imageManager.requestImage(for: asset, targetSize: CGSize(width: asset.pixelWidth, height: asset.pixelHeight), contentMode: .aspectFit, options: settings.fetch.preview.photoOptions) { [weak self] (image, _) in
guard let image = image else { return }
self?.imageView.image = image
}
}
}
let imageView: UIImageView = UIImageView(frame: .zero)
var fullscreen = false {
didSet {
guard oldValue != fullscreen else { return }
UIView.animate(withDuration: 0.3) {
self.updateNavigationBar()
self.updateStatusBar()
self.updateBackgroundColor()
}
}
}
let scrollView = UIScrollView(frame: .zero)
let singleTapRecognizer = UITapGestureRecognizer()
let doubleTapRecognizer = UITapGestureRecognizer()
private let titleLabel = UILabel(frame: .zero)
override var prefersStatusBarHidden : Bool {
return fullscreen
}
required init() {
super.init(nibName: nil, bundle: nil)
setupScrollView()
setupImageView()
setupSingleTapRecognizer()
setupDoubleTapRecognizer()
}
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
private func setupScrollView() {
scrollView.frame = view.bounds
scrollView.autoresizingMask = [.flexibleWidth, .flexibleHeight]
scrollView.delegate = self
scrollView.minimumZoomScale = 1
scrollView.maximumZoomScale = 3
if #available(iOS 11.0, *) {
// Allows the imageview to be 'under' the navigation bar
scrollView.contentInsetAdjustmentBehavior = .never
}
scrollView.showsVerticalScrollIndicator = false
scrollView.showsHorizontalScrollIndicator = false
view.addSubview(scrollView)
}
private func setupImageView() {
imageView.frame = scrollView.bounds
imageView.autoresizingMask = [.flexibleWidth, .flexibleHeight]
imageView.contentMode = .scaleAspectFit
scrollView.addSubview(imageView)
}
private func setupSingleTapRecognizer() {
singleTapRecognizer.numberOfTapsRequired = 1
singleTapRecognizer.addTarget(self, action: #selector(didSingleTap(_:)))
singleTapRecognizer.require(toFail: doubleTapRecognizer)
view.addGestureRecognizer(singleTapRecognizer)
}
private func setupDoubleTapRecognizer() {
doubleTapRecognizer.numberOfTapsRequired = 2
doubleTapRecognizer.addTarget(self, action: #selector(didDoubleTap(_:)))
view.addGestureRecognizer(doubleTapRecognizer)
}
private func setupTitleLabel() {
titleLabel.numberOfLines = 0
titleLabel.lineBreakMode = .byClipping
titleLabel.textAlignment = .center
navigationItem.titleView = titleLabel
}
override func viewDidLoad() {
super.viewDidLoad()
updateBackgroundColor()
setupTitleLabel()
}
override func viewWillDisappear(_ animated: Bool) {
super.viewWillDisappear(animated)
fullscreen = false
}
private func toggleFullscreen() {
fullscreen = !fullscreen
}
@objc func didSingleTap(_ recognizer: UIGestureRecognizer) {
toggleFullscreen()
}
@objc func didDoubleTap(_ recognizer: UIGestureRecognizer) {
if scrollView.zoomScale > 1 {
scrollView.setZoomScale(1, animated: true)
} else {
scrollView.zoom(to: zoomRect(scale: 2, center: recognizer.location(in: recognizer.view)), animated: true)
}
}
private func zoomRect(scale: CGFloat, center: CGPoint) -> CGRect {
guard let zoomView = viewForZooming(in: scrollView) else { return .zero }
let newCenter = scrollView.convert(center, from: zoomView)
var zoomRect = CGRect.zero
zoomRect.size.height = zoomView.frame.size.height / scale
zoomRect.size.width = zoomView.frame.size.width / scale
zoomRect.origin.x = newCenter.x - (zoomRect.size.width / 2.0)
zoomRect.origin.y = newCenter.y - (zoomRect.size.height / 2.0)
return zoomRect
}
private func updateNavigationBar() {
navigationController?.setNavigationBarHidden(fullscreen, animated: true)
}
private func updateStatusBar() {
self.setNeedsStatusBarAppearanceUpdate()
}
private func updateBackgroundColor() {
let aColor: UIColor
if self.fullscreen && modalPresentationStyle == .fullScreen {
aColor = UIColor.black
} else {
aColor = UIColor.systemBackgroundColor
}
view.backgroundColor = aColor
}
private func updateNavigationTitle() {
guard let asset = asset else { return }
PreviewTitleBuilder.titleFor(asset: asset,using:settings.theme) { [weak self] (text) in
self?.titleLabel.attributedText = text
self?.titleLabel.sizeToFit()
}
}
}
extension PreviewViewController: UIScrollViewDelegate {
func viewForZooming(in scrollView: UIScrollView) -> UIView? {
return imageView
}
func scrollViewDidZoom(_ scrollView: UIScrollView) {
if scrollView.zoomScale > 1 {
fullscreen = true
guard let image = imageView.image else { return }
guard let zoomView = viewForZooming(in: scrollView) else { return }
let widthRatio = zoomView.frame.width / image.size.width
let heightRatio = zoomView.frame.height / image.size.height
let ratio = widthRatio < heightRatio ? widthRatio:heightRatio
let newWidth = image.size.width * ratio
let newHeight = image.size.height * ratio
let left = 0.5 * (newWidth * scrollView.zoomScale > zoomView.frame.width ? (newWidth - zoomView.frame.width) : (scrollView.frame.width - scrollView.contentSize.width))
let top = 0.5 * (newHeight * scrollView.zoomScale > zoomView.frame.height ? (newHeight - zoomView.frame.height) : (scrollView.frame.height - scrollView.contentSize.height))
scrollView.contentInset = UIEdgeInsets(top: top.rounded(), left: left.rounded(), bottom: top.rounded(), right: left.rounded())
} else {
scrollView.contentInset = .zero
}
}
}
| mit | 06f367cc014c24adad1dc9b77803defa | 35.352941 | 220 | 0.655962 | 5.299472 | false | false | false | false |
optimizely/swift-sdk | Sources/Optimizely/OptimizelyJSON.swift | 1 | 7465 | /****************************************************************************
* Copyright 2020-2021, Optimizely, Inc. and contributors *
* *
* Licensed under the Apache License, Version 2.0 (the "License"); *
* you may not use this file except in compliance with the License. *
* You may obtain a copy of the License at *
* *
* http://www.apache.org/licenses/LICENSE-2.0 *
* *
* Unless required by applicable law or agreed to in writing, software *
* distributed under the License is distributed on an "AS IS" BASIS, *
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. *
* See the License for the specific language governing permissions and *
* limitations under the License. *
***************************************************************************/
import Foundation
public class OptimizelyJSON: NSObject {
private typealias ValueHandler<T> = (Any) -> T?
var payload: String?
var map = [String: Any]()
let logger = OPTLoggerFactory.getLogger()
// MARK: - Init
init?(payload: String) {
do {
guard let data = payload.data(using: .utf8),
let dict = try JSONSerialization.jsonObject(with: data, options: .allowFragments) as? [String: Any] else {
return nil
}
self.map = dict
self.payload = payload
} catch {
return nil
}
}
init?(map: [String: Any]) {
if !JSONSerialization.isValidJSONObject(map) {
return nil
}
self.map = map
}
static func createEmpty() -> OptimizelyJSON {
return OptimizelyJSON(map: [:])!
}
public var isEmpty: Bool {
return map.isEmpty
}
// MARK: - OptimizelyJSON Implementation
/// - Returns: The string representation of json
public func toString() -> String? {
guard let payload = self.payload else {
guard let jsonData = try? JSONSerialization.data(withJSONObject: map,
options: []),
let jsonString = String(data: jsonData, encoding: .utf8) else {
logger.e(.failedToConvertMapToString)
return nil
}
self.payload = jsonString
return jsonString
}
return payload
}
/// - Returns: The json dictionary
public func toMap() -> [String: Any] {
return map
}
/// Returns decoded value for jsonPath
///
/// If JSON Data is {"k1":true, "k2":{"k3":"v3"}}
///
/// Set jsonPath to "k2" to access {"k3":"v3"} or set it to "k2.k3" to access "v3".
/// Set it to nil or empty to access the entire JSON data. See more examples below:
///
///
/// struct Student: Decodable {
/// let name: String
/// let age: Int
/// let address: Address
/// }
///
/// struct Address: Decodable {
/// let state: String
/// let emails: [String]
/// }
///
/// let student: Student? = optimizelyJSON.getValue(jsonPath: nil)
/// let address: Address? = optimizelyJSON.getValue(jsonPath: "address")
/// let name: String? = optimizelyJSON.getValue(jsonPath: "name")
/// let emails: [String]? = optimizelyJSON.getValue(jsonPath: "address.emails")
///
///
/// - Parameters:
/// - jsonPath: Key path for the value.
/// - Returns: Value if decoded successfully
public func getValue<T: Decodable>(jsonPath: String?) -> T? {
func handler(value: Any) -> T? {
guard JSONSerialization.isValidJSONObject(value) else {
// Try and typecast value to required return type
if let v = value as? T {
return v
}
logger.e(.failedToAssignValue)
return nil
}
// Try to decode value into return type
guard let jsonData = try? JSONSerialization.data(withJSONObject: value, options: []),
let decodedValue = try? JSONDecoder().decode(T.self, from: jsonData) else {
logger.e(.failedToAssignValue)
return nil
}
return decodedValue
}
return getValue(jsonPath: jsonPath, valueHandler: handler(value:))
}
/// Returns parsed value for jsonPath
///
/// If JSON Data is {"k1":true, "k2":{"k3":"v3"}}
///
/// Set jsonPath to "k2" to access {"k3":"v3"} or set it to "k2.k3" to access "v3".
/// Set it to nil or empty to access the entire JSON data. See more examples below:
///
///
/// struct Student: Decodable {
/// let name: String
/// let age: Int
/// let address: Address
/// }
///
/// struct Address: Decodable {
/// let state: String
/// let emails: [String]
/// }
///
/// let student: Student? = optimizelyJSON.getValue(jsonPath: nil)
/// let address: Address? = optimizelyJSON.getValue(jsonPath: "address")
/// let name: String? = optimizelyJSON.getValue(jsonPath: "name")
/// let emails: [String]? = optimizelyJSON.getValue(jsonPath: "address.emails")
///
///
/// - Parameters:
/// - jsonPath: Key path for the value.
/// - Returns: Value if parsed successfully
public func getValue<T>(jsonPath: String?) -> T? {
func handler(value: Any) -> T? {
guard let v = value as? T else {
self.logger.e(.failedToAssignValue)
return nil
}
return v
}
return getValue(jsonPath: jsonPath, valueHandler: handler(value:))
}
private func getValue<T>(jsonPath: String?, valueHandler: ValueHandler<T>) -> T? {
guard let path = jsonPath, !path.isEmpty else {
// Retrieve value for path
return valueHandler(map)
}
let pathArray = path.components(separatedBy: ".")
let lastIndex = pathArray.count - 1
var internalMap = map
for (index, key) in pathArray.enumerated() {
guard let value = internalMap[key] else {
self.logger.e(.valueForKeyNotFound(key))
return nil
}
if let dict = value as? [String: Any] {
internalMap = dict
}
if index == lastIndex {
return valueHandler(value)
}
}
return nil
}
}
extension OptimizelyJSON {
// override NSObject Equatable ('==' overriding not working for NSObject)
override public func isEqual(_ object: Any?) -> Bool {
guard let object = object as? OptimizelyJSON else { return false }
return NSDictionary(dictionary: map).isEqual(to: object.toMap())
}
}
extension OptimizelyJSON {
public override var description: String {
return "\(map)"
}
}
| apache-2.0 | 1dff49e234f73e40c405f7a251164853 | 35.062802 | 122 | 0.508372 | 4.911184 | false | false | false | false |
VirgilSecurity/virgil-sdk-pfs-x | Source/Utils/Log.swift | 1 | 1028 | //
// Log.swift
// VirgilSDKPFS
//
// Created by Oleksandr Deundiak on 8/23/17.
// Copyright © 2017 VirgilSecurity. All rights reserved.
//
class Log {
class func debug(_ closure: @autoclosure () -> String, functionName: String = #function, file: String = #file, line: UInt = #line) {
#if DEBUG
self.log("<DEBUG>: \(closure())", functionName: functionName, file: file, line: line)
#endif
}
class func error( _ closure: @autoclosure () -> String, functionName: String = #function, file: String = #file, line: UInt = #line) {
self.log("<ERROR>: \(closure())", functionName: functionName, file: file, line: line)
}
private class func log(_ closure: @autoclosure () -> String, functionName: String = #function, file: String = #file, line: UInt = #line) {
let str = "PFS_LOG: \(functionName) : \(closure())"
Log.writeInLog(str)
}
private class func writeInLog(_ message: String) {
NSLogv("%@", getVaList([message]))
}
}
| bsd-3-clause | f587e3505ca8be5d4bcf0911fa785ce5 | 35.678571 | 142 | 0.601753 | 3.875472 | false | false | false | false |
yanif/circator | MetabolicCompass/DataSources/ChartCollectionDataSource.swift | 1 | 2941 | //
// ChartCollectionDataSource.swift
// ChartsMC
//
// Created by Artem Usachov on 6/1/16.
// Copyright © 2016 SROST. All rights reserved.
//
import Foundation
import UIKit
import Charts
import HealthKit
import MetabolicCompassKit
class ChartCollectionDataSource: NSObject, UICollectionViewDataSource {
internal var collectionData: [ChartData] = []
internal var model: BarChartModel?
internal var data: [HKSampleType] = PreviewManager.chartsSampleTypes
private let appearanceProvider = DashboardMetricsAppearanceProvider()
private let barChartCellIdentifier = "BarChartCollectionCell"
private let lineChartCellIdentifier = "LineChartCollectionCell"
private let scatterChartCellIdentifier = "ScatterChartCollectionCell"
func updateData () {
data = PreviewManager.chartsSampleTypes
}
func collectionView(collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int {
return data.count
}
func collectionView(collectionView: UICollectionView, cellForItemAtIndexPath indexPath: NSIndexPath) -> UICollectionViewCell {
let cell: BaseChartCollectionCell
let type = data[indexPath.row]
let typeToShow = type.identifier == HKCorrelationTypeIdentifierBloodPressure ? HKQuantityTypeIdentifierBloodPressureSystolic : type.identifier
let chartType: ChartType = (model?.chartTypeForQuantityTypeIdentifier(typeToShow))!
let key = typeToShow + "\((model?.rangeType.rawValue)!)"
let chartData = model?.typesChartData[key]
if(chartType == ChartType.BarChart) {
cell = collectionView.dequeueReusableCellWithReuseIdentifier(barChartCellIdentifier, forIndexPath: indexPath) as! BarChartCollectionCell
} else if (chartType == ChartType.LineChart) {
cell = collectionView.dequeueReusableCellWithReuseIdentifier(lineChartCellIdentifier, forIndexPath: indexPath) as! LineChartCollectionCell
} else {//Scatter chart
cell = collectionView.dequeueReusableCellWithReuseIdentifier(scatterChartCellIdentifier, forIndexPath: indexPath) as! ScatterChartCollectionCell
}
cell.chartView.data = nil
if let yMax = chartData?.yMax, yMin = chartData?.yMin where yMax > 0 || yMin > 0 {
cell.updateLeftAxisWith(chartData?.yMin, maxValue: chartData?.yMax)
cell.chartView.data = chartData
if let marker = cell.chartView.marker as? BalloonMarker {
marker.yMax = cell.chartView.leftAxis.axisMaxValue
marker.yMin = cell.chartView.leftAxis.axisMinValue
marker.yPixelRange = Double(cell.chartView.contentRect.height)
}
}
cell.chartTitleLabel.text = appearanceProvider.stringForSampleType(typeToShow == HKQuantityTypeIdentifierBloodPressureSystolic ? HKCorrelationTypeIdentifierBloodPressure : typeToShow)
return cell
}
} | apache-2.0 | eafa83a47015f6ee613ea93fbd9b1c19 | 47.213115 | 191 | 0.730272 | 5.568182 | false | false | false | false |
annatovstyga/REA-Schedule | Raspisaniye/Data Model/OneLesson.swift | 1 | 2045 | //
// OneLesson.swift
// Raspisaniye
//
// Created by Ilya Mudriy on 10.02.16.
// Copyright © 2016 rGradeStd. All rights reserved.
//
import Foundation
class OneLesson {
var lessonNumber: Int?
var hashID: String?
var lessonType: String?
var room: String?
var lessonStart: String?
var lessonEnd: String?
var discipline: String?
var building: String?
var lector: String?
var house: Int?
var groups: [String]?
var startWeek: Int?
var endWeek: Int?
// MARK: - Initializators
init() {}
init(lessonNumber: Int?, hashID: String?, lessonType: String?, room: String?, lessonStart: String?, lessonEnd: String?, discipline: String?, building: String?, lector: String?, house: Int?, groups: [String]?,startWeek:Int?,endWeek:Int?) {
self.lessonNumber = lessonNumber
self.hashID = hashID
self.lessonType = lessonType
self.room = room
self.lessonStart = lessonStart
self.lessonEnd = lessonEnd
self.discipline = discipline
self.building = building
self.lector = lector
self.house = house
self.groups = groups
self.startWeek = startWeek
self.endWeek = endWeek
}
// MARK: - Clear method
func clearAll() {
self.lessonNumber = nil
self.hashID = nil
self.lessonType = nil
self.room = nil
self.lessonStart = nil
self.lessonEnd = nil
self.discipline = nil
self.building = nil
self.lector = nil
self.house = nil
self.groups = nil
}
// MARK: - Print in log
func description() {
print("\nlessonNumber - \(lessonNumber)\nhashID - \(hashID)\nlessonType - \(lessonType)\nroom - \(room)\nlessonStart - \(lessonStart)\nlessonEnd - \(lessonEnd)\ndiscipline - \(discipline)\nbuilding - \(building)\nlector - \(lector)\nhouse - \(house)\ngroups - \(groups)")
}
} | mit | 84325a1c9bcb8e6d156b90b25607efd8 | 29.522388 | 279 | 0.58317 | 3.984405 | false | false | false | false |
StevenPreston/webview-utilities | Example/Tests/Pod/WKWebView+UtilitiesSpec.swift | 1 | 4646 | //
// WKWebView+UtilitiesSpec.swift
// WebView-Utilities
//
// Created by Steven Preston on 9/8/16.
// Copyright © 2016 CocoaPods. All rights reserved.
//
import Quick
import Nimble
@testable import WebView_Utilities
import WebKit
class NavigationDelegate: NSObject, WKNavigationDelegate {
var pageLoaded = false
@objc func webView(webView: WKWebView, didFinishNavigation navigation: WKNavigation!) {
pageLoaded = true
}
}
struct WebResult {
var noError: Bool
var response: Bool
init() {
self.noError = false
self.response = false
}
init(response: Bool, noError: Bool) {
self.noError = noError
self.response = response
}
}
class WKWebView_UtilitiesSpec: QuickSpec {
override func spec() {
let webView = WKWebView(frame: CGRectMake(0, 0, 320, 640))
let navigationDelegate = NavigationDelegate()
let html =
"<!DOCTYPE html>" +
"<html>" +
" <head></head>" +
" <body>" +
" <div id='keep'>keep</div>" +
" <div id='remove'>remove</div>" +
" </body>" +
"</html>"
var webResult = WebResult()
let resultHandler = { (result: AnyObject?, error: NSError?) -> WebResult in
let noError = error == nil
var response = false
if let tempResult = result as? Bool {
response = tempResult
}
return WebResult(response: response, noError: noError)
}
beforeEach {
webResult = WebResult()
navigationDelegate.pageLoaded = false
webView.navigationDelegate = navigationDelegate
webView.loadHTMLString(html, baseURL: nil)
expect(navigationDelegate.pageLoaded).toEventually(beTrue())
}
describe("clearHeadJS") {
it("should return a Javascript value of true") {
webView.clearHead() { webResult = resultHandler($0, $1) }
expect(webResult.noError).toEventually(beTrue())
expect(webResult.response).to(beTrue())
}
}
describe("WKWebView.stripAllOtherElementsFromBody") {
context("when the element to keep exists") {
it("should return a Javascript value of true") {
webView.stripAllOtherElementsFromBody("keep") { webResult = resultHandler($0, $1) }
expect(webResult.noError).toEventually(beTrue())
expect(webResult.response).to(beTrue())
}
}
context("when the element to keep does not exist") {
it("should return a Javascript value of true") {
webView.stripAllOtherElementsFromBody("nonexistent") { webResult = resultHandler($0, $1) }
expect(webResult.noError).toEventually(beTrue())
expect(webResult.response).to(beFalse())
}
}
}
describe("WKWebView.addElementToHead") {
it("should return a Javascript value of true") {
let elementHTML = "<style>body{margin: 20px;}</style>"
webView.addElementToHead(elementHTML) { webResult = resultHandler($0, $1) }
expect(webResult.noError).toEventually(beTrue())
expect(webResult.response).to(beTrue())
}
}
describe("WKWebView.removeElement") {
context("when the element to keep exists") {
it("should return a Javascript value of true") {
webView.removeElement("remove") { webResult = resultHandler($0, $1) }
expect(webResult.noError).toEventually(beTrue())
expect(webResult.response).to(beTrue())
}
}
context("when the element to keep does not exist") {
it("should return a Javascript value of true") {
webView.removeElement("nonexistent") { webResult = resultHandler($0, $1) }
expect(webResult.noError).toEventually(beTrue())
expect(webResult.response).to(beFalse())
}
}
}
describe("WKWebView.addCSS") {
it("should return a Javascript value of true") {
let css = "body{margin: 20px;}"
webView.addCSS(css) { webResult = resultHandler($0, $1) }
expect(webResult.noError).toEventually(beTrue())
expect(webResult.response).to(beTrue())
}
}
}
}
| mit | 6489f41b3c34ea4bc4069b8a4e6f46fa | 34.730769 | 110 | 0.550915 | 5.010787 | false | false | false | false |
faraxnak/PayWandBasicElements | Pods/SwiftyButton/SwiftyButton/FlatButton.swift | 2 | 2884 | //
// FlatButton.swift
// SwiftyButton
//
// Created by Lois Di Qual on 10/23/16.
// Copyright © 2016 TakeScoop. All rights reserved.
//
import UIKit
@IBDesignable
public class FlatButton: UIButton {
public enum Defaults {
public static var color = UIColor(red: 52 / 255, green: 152 / 255, blue: 219 / 255, alpha: 1)
public static var highlightedColor = UIColor(red: 41 / 255, green: 128 / 255, blue: 185 / 255, alpha: 1)
public static var selectedColor = UIColor(red: 41 / 255, green: 128 / 255, blue: 185 / 255, alpha: 1)
public static var disabledColor = UIColor(red: 149 / 255, green: 165 / 255, blue: 166 / 255, alpha: 1)
public static var cornerRadius: CGFloat = 3
}
@IBInspectable
public var color: UIColor = Defaults.color {
didSet {
updateBackgroundImages()
}
}
@IBInspectable
public var highlightedColor: UIColor = Defaults.highlightedColor {
didSet {
updateBackgroundImages()
}
}
@IBInspectable
public var selectedColor: UIColor = Defaults.selectedColor {
didSet {
updateBackgroundImages()
}
}
@IBInspectable
public var disabledColor: UIColor = Defaults.disabledColor {
didSet {
updateBackgroundImages()
}
}
@IBInspectable
public var cornerRadius: CGFloat = Defaults.cornerRadius {
didSet {
updateBackgroundImages()
}
}
// MARK: - UIButton
public override init(frame: CGRect) {
super.init(frame: frame)
configure()
updateBackgroundImages()
}
public required init?(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)
configure()
updateBackgroundImages()
}
// MARK: - Internal methods
fileprivate func configure() {
adjustsImageWhenDisabled = false
adjustsImageWhenHighlighted = false
}
fileprivate func updateBackgroundImages() {
let normalImage = Utils.buttonImage(color: color, shadowHeight: 0, shadowColor: .clear, cornerRadius: cornerRadius)
let highlightedImage = Utils.highlightedButtonImage(color: highlightedColor, shadowHeight: 0, shadowColor: .clear, cornerRadius: cornerRadius, buttonPressDepth: 0)
let selectedImage = Utils.buttonImage(color: selectedColor, shadowHeight: 0, shadowColor: .clear, cornerRadius: cornerRadius)
let disabledImage = Utils.buttonImage(color: disabledColor, shadowHeight: 0, shadowColor: .clear, cornerRadius: cornerRadius)
setBackgroundImage(normalImage, for: .normal)
setBackgroundImage(highlightedImage, for: .highlighted)
setBackgroundImage(selectedImage, for: .selected)
setBackgroundImage(disabledImage, for: .disabled)
}
}
| mit | 0ffec85d1e36a548a7d98c900e6c8e14 | 31.033333 | 171 | 0.640305 | 5.066784 | false | false | false | false |
leizh007/HiPDA | HiPDA/HiPDA/Sections/Message/SendShortMessage/SendShortMessageViewModel.swift | 1 | 1620 | //
// SendShortMessageViewModel.swift
// HiPDA
//
// Created by leizh007 on 2017/6/26.
// Copyright © 2017年 HiPDA. All rights reserved.
//
import Foundation
import RxSwift
class SendShortMessageViewModel {
private var disposeBag = DisposeBag()
let user: User
init(user: User) {
self.user = user
}
func sendMessage(_ message: String, completion: @escaping (HiPDA.Result<String, NSError>) -> Void) {
disposeBag = DisposeBag()
NetworkUtilities.formhash(from: "/forum/pm.php?action=new&uid=\(user.uid)") { [weak self] result in
guard let `self` = self else { return }
switch result {
case .failure(let error):
completion(.failure(error as NSError))
case .success(let formhash):
HiPDAProvider.request(.sendShortMessage(username: self.user.name, message: message, formhash: formhash))
.observeOn(ConcurrentDispatchQueueScheduler(qos: .userInteractive))
.mapGBKString()
.map { return try HtmlParser.alertInfo(from: $0) }
.observeOn(MainScheduler.instance).subscribe { event in
switch event {
case .next(let info):
completion(.success(info))
case .error(let error):
completion(.failure(error as NSError))
default:
break
}
}.disposed(by: self.disposeBag)
}
}
}
}
| mit | 824bc91bd0b0501b14cb3f8563d9882b | 35.75 | 120 | 0.536797 | 4.990741 | false | false | false | false |
milseman/swift | stdlib/public/SDK/Foundation/JSONEncoder.swift | 4 | 95156 | //===----------------------------------------------------------------------===//
//
// This source file is part of the Swift.org open source project
//
// Copyright (c) 2014 - 2017 Apple Inc. and the Swift project authors
// Licensed under Apache License v2.0 with Runtime Library Exception
//
// See https://swift.org/LICENSE.txt for license information
// See https://swift.org/CONTRIBUTORS.txt for the list of Swift project authors
//
//===----------------------------------------------------------------------===//
//===----------------------------------------------------------------------===//
// JSON Encoder
//===----------------------------------------------------------------------===//
/// `JSONEncoder` facilitates the encoding of `Encodable` values into JSON.
open class JSONEncoder {
// MARK: Options
/// The formatting of the output JSON data.
public struct OutputFormatting : OptionSet {
/// The format's default value.
public let rawValue: UInt
/// Creates an OutputFormatting value with the given raw value.
public init(rawValue: UInt) {
self.rawValue = rawValue
}
/// Produce human-readable JSON with indented output.
public static let prettyPrinted = OutputFormatting(rawValue: 1 << 0)
/// Produce JSON with dictionary keys sorted in lexicographic order.
@available(OSX 10.13, iOS 11.0, watchOS 4.0, tvOS 11.0, *)
public static let sortedKeys = OutputFormatting(rawValue: 1 << 1)
}
/// The strategy to use for encoding `Date` values.
public enum DateEncodingStrategy {
/// Defer to `Date` for choosing an encoding. This is the default strategy.
case deferredToDate
/// Encode the `Date` as a UNIX timestamp (as a JSON number).
case secondsSince1970
/// Encode the `Date` as UNIX millisecond timestamp (as a JSON number).
case millisecondsSince1970
/// Encode the `Date` as an ISO-8601-formatted string (in RFC 3339 format).
@available(OSX 10.12, iOS 10.0, watchOS 3.0, tvOS 10.0, *)
case iso8601
/// Encode the `Date` as a string formatted by the given formatter.
case formatted(DateFormatter)
/// Encode the `Date` as a custom value encoded by the given closure.
///
/// If the closure fails to encode a value into the given encoder, the encoder will encode an empty automatic container in its place.
case custom((Date, Encoder) throws -> Void)
}
/// The strategy to use for encoding `Data` values.
public enum DataEncodingStrategy {
/// Defer to `Data` for choosing an encoding.
case deferredToData
/// Encoded the `Data` as a Base64-encoded string. This is the default strategy.
case base64
/// Encode the `Data` as a custom value encoded by the given closure.
///
/// If the closure fails to encode a value into the given encoder, the encoder will encode an empty automatic container in its place.
case custom((Data, Encoder) throws -> Void)
}
/// The strategy to use for non-JSON-conforming floating-point values (IEEE 754 infinity and NaN).
public enum NonConformingFloatEncodingStrategy {
/// Throw upon encountering non-conforming values. This is the default strategy.
case `throw`
/// Encode the values using the given representation strings.
case convertToString(positiveInfinity: String, negativeInfinity: String, nan: String)
}
/// The output format to produce. Defaults to `[]`.
open var outputFormatting: OutputFormatting = []
/// The strategy to use in encoding dates. Defaults to `.deferredToDate`.
open var dateEncodingStrategy: DateEncodingStrategy = .deferredToDate
/// The strategy to use in encoding binary data. Defaults to `.base64`.
open var dataEncodingStrategy: DataEncodingStrategy = .base64
/// The strategy to use in encoding non-conforming numbers. Defaults to `.throw`.
open var nonConformingFloatEncodingStrategy: NonConformingFloatEncodingStrategy = .throw
/// Contextual user-provided information for use during encoding.
open var userInfo: [CodingUserInfoKey : Any] = [:]
/// Options set on the top-level encoder to pass down the encoding hierarchy.
fileprivate struct _Options {
let dateEncodingStrategy: DateEncodingStrategy
let dataEncodingStrategy: DataEncodingStrategy
let nonConformingFloatEncodingStrategy: NonConformingFloatEncodingStrategy
let userInfo: [CodingUserInfoKey : Any]
}
/// The options set on the top-level encoder.
fileprivate var options: _Options {
return _Options(dateEncodingStrategy: dateEncodingStrategy,
dataEncodingStrategy: dataEncodingStrategy,
nonConformingFloatEncodingStrategy: nonConformingFloatEncodingStrategy,
userInfo: userInfo)
}
// MARK: - Constructing a JSON Encoder
/// Initializes `self` with default strategies.
public init() {}
// MARK: - Encoding Values
/// Encodes the given top-level value and returns its JSON representation.
///
/// - parameter value: The value to encode.
/// - returns: A new `Data` value containing the encoded JSON data.
/// - throws: `EncodingError.invalidValue` if a non-conforming floating-point value is encountered during encoding, and the encoding strategy is `.throw`.
/// - throws: An error if any value throws an error during encoding.
open func encode<T : Encodable>(_ value: T) throws -> Data {
let encoder = _JSONEncoder(options: self.options)
try value.encode(to: encoder)
guard encoder.storage.count > 0 else {
throw EncodingError.invalidValue(value, EncodingError.Context(codingPath: [], debugDescription: "Top-level \(T.self) did not encode any values."))
}
let topLevel = encoder.storage.popContainer()
if topLevel is NSNull {
throw EncodingError.invalidValue(value, EncodingError.Context(codingPath: [], debugDescription: "Top-level \(T.self) encoded as null JSON fragment."))
} else if topLevel is NSNumber {
throw EncodingError.invalidValue(value, EncodingError.Context(codingPath: [], debugDescription: "Top-level \(T.self) encoded as number JSON fragment."))
} else if topLevel is NSString {
throw EncodingError.invalidValue(value, EncodingError.Context(codingPath: [], debugDescription: "Top-level \(T.self) encoded as string JSON fragment."))
}
let writingOptions = JSONSerialization.WritingOptions(rawValue: self.outputFormatting.rawValue)
do {
return try JSONSerialization.data(withJSONObject: topLevel, options: writingOptions)
} catch {
throw EncodingError.invalidValue(value, EncodingError.Context(codingPath: [], debugDescription: "Unable to encode the given top-level value to JSON.", underlyingError: error))
}
}
}
// MARK: - _JSONEncoder
fileprivate class _JSONEncoder : Encoder {
// MARK: Properties
/// The encoder's storage.
fileprivate var storage: _JSONEncodingStorage
/// Options set on the top-level encoder.
fileprivate let options: JSONEncoder._Options
/// The path to the current point in encoding.
public var codingPath: [CodingKey]
/// Contextual user-provided information for use during encoding.
public var userInfo: [CodingUserInfoKey : Any] {
return self.options.userInfo
}
// MARK: - Initialization
/// Initializes `self` with the given top-level encoder options.
fileprivate init(options: JSONEncoder._Options, codingPath: [CodingKey] = []) {
self.options = options
self.storage = _JSONEncodingStorage()
self.codingPath = codingPath
}
/// Returns whether a new element can be encoded at this coding path.
///
/// `true` if an element has not yet been encoded at this coding path; `false` otherwise.
fileprivate var canEncodeNewValue: Bool {
// Every time a new value gets encoded, the key it's encoded for is pushed onto the coding path (even if it's a nil key from an unkeyed container).
// At the same time, every time a container is requested, a new value gets pushed onto the storage stack.
// If there are more values on the storage stack than on the coding path, it means the value is requesting more than one container, which violates the precondition.
//
// This means that anytime something that can request a new container goes onto the stack, we MUST push a key onto the coding path.
// Things which will not request containers do not need to have the coding path extended for them (but it doesn't matter if it is, because they will not reach here).
return self.storage.count == self.codingPath.count
}
// MARK: - Encoder Methods
public func container<Key>(keyedBy: Key.Type) -> KeyedEncodingContainer<Key> {
// If an existing keyed container was already requested, return that one.
let topContainer: NSMutableDictionary
if self.canEncodeNewValue {
// We haven't yet pushed a container at this level; do so here.
topContainer = self.storage.pushKeyedContainer()
} else {
guard let container = self.storage.containers.last as? NSMutableDictionary else {
preconditionFailure("Attempt to push new keyed encoding container when already previously encoded at this path.")
}
topContainer = container
}
let container = _JSONKeyedEncodingContainer<Key>(referencing: self, codingPath: self.codingPath, wrapping: topContainer)
return KeyedEncodingContainer(container)
}
public func unkeyedContainer() -> UnkeyedEncodingContainer {
// If an existing unkeyed container was already requested, return that one.
let topContainer: NSMutableArray
if self.canEncodeNewValue {
// We haven't yet pushed a container at this level; do so here.
topContainer = self.storage.pushUnkeyedContainer()
} else {
guard let container = self.storage.containers.last as? NSMutableArray else {
preconditionFailure("Attempt to push new unkeyed encoding container when already previously encoded at this path.")
}
topContainer = container
}
return _JSONUnkeyedEncodingContainer(referencing: self, codingPath: self.codingPath, wrapping: topContainer)
}
public func singleValueContainer() -> SingleValueEncodingContainer {
return self
}
}
// MARK: - Encoding Storage and Containers
fileprivate struct _JSONEncodingStorage {
// MARK: Properties
/// The container stack.
/// Elements may be any one of the JSON types (NSNull, NSNumber, NSString, NSArray, NSDictionary).
private(set) fileprivate var containers: [NSObject] = []
// MARK: - Initialization
/// Initializes `self` with no containers.
fileprivate init() {}
// MARK: - Modifying the Stack
fileprivate var count: Int {
return self.containers.count
}
fileprivate mutating func pushKeyedContainer() -> NSMutableDictionary {
let dictionary = NSMutableDictionary()
self.containers.append(dictionary)
return dictionary
}
fileprivate mutating func pushUnkeyedContainer() -> NSMutableArray {
let array = NSMutableArray()
self.containers.append(array)
return array
}
fileprivate mutating func push(container: NSObject) {
self.containers.append(container)
}
fileprivate mutating func popContainer() -> NSObject {
precondition(self.containers.count > 0, "Empty container stack.")
return self.containers.popLast()!
}
}
// MARK: - Encoding Containers
fileprivate struct _JSONKeyedEncodingContainer<K : CodingKey> : KeyedEncodingContainerProtocol {
typealias Key = K
// MARK: Properties
/// A reference to the encoder we're writing to.
private let encoder: _JSONEncoder
/// A reference to the container we're writing to.
private let container: NSMutableDictionary
/// The path of coding keys taken to get to this point in encoding.
private(set) public var codingPath: [CodingKey]
// MARK: - Initialization
/// Initializes `self` with the given references.
fileprivate init(referencing encoder: _JSONEncoder, codingPath: [CodingKey], wrapping container: NSMutableDictionary) {
self.encoder = encoder
self.codingPath = codingPath
self.container = container
}
// MARK: - KeyedEncodingContainerProtocol Methods
public mutating func encodeNil(forKey key: Key) throws { self.container[key.stringValue] = NSNull() }
public mutating func encode(_ value: Bool, forKey key: Key) throws { self.container[key.stringValue] = self.encoder.box(value) }
public mutating func encode(_ value: Int, forKey key: Key) throws { self.container[key.stringValue] = self.encoder.box(value) }
public mutating func encode(_ value: Int8, forKey key: Key) throws { self.container[key.stringValue] = self.encoder.box(value) }
public mutating func encode(_ value: Int16, forKey key: Key) throws { self.container[key.stringValue] = self.encoder.box(value) }
public mutating func encode(_ value: Int32, forKey key: Key) throws { self.container[key.stringValue] = self.encoder.box(value) }
public mutating func encode(_ value: Int64, forKey key: Key) throws { self.container[key.stringValue] = self.encoder.box(value) }
public mutating func encode(_ value: UInt, forKey key: Key) throws { self.container[key.stringValue] = self.encoder.box(value) }
public mutating func encode(_ value: UInt8, forKey key: Key) throws { self.container[key.stringValue] = self.encoder.box(value) }
public mutating func encode(_ value: UInt16, forKey key: Key) throws { self.container[key.stringValue] = self.encoder.box(value) }
public mutating func encode(_ value: UInt32, forKey key: Key) throws { self.container[key.stringValue] = self.encoder.box(value) }
public mutating func encode(_ value: UInt64, forKey key: Key) throws { self.container[key.stringValue] = self.encoder.box(value) }
public mutating func encode(_ value: String, forKey key: Key) throws { self.container[key.stringValue] = self.encoder.box(value) }
public mutating func encode(_ value: Float, forKey key: Key) throws {
// Since the float may be invalid and throw, the coding path needs to contain this key.
self.encoder.codingPath.append(key)
defer { self.encoder.codingPath.removeLast() }
self.container[key.stringValue] = try self.encoder.box(value)
}
public mutating func encode(_ value: Double, forKey key: Key) throws {
// Since the double may be invalid and throw, the coding path needs to contain this key.
self.encoder.codingPath.append(key)
defer { self.encoder.codingPath.removeLast() }
self.container[key.stringValue] = try self.encoder.box(value)
}
public mutating func encode<T : Encodable>(_ value: T, forKey key: Key) throws {
self.encoder.codingPath.append(key)
defer { self.encoder.codingPath.removeLast() }
self.container[key.stringValue] = try self.encoder.box(value)
}
public mutating func nestedContainer<NestedKey>(keyedBy keyType: NestedKey.Type, forKey key: Key) -> KeyedEncodingContainer<NestedKey> {
let dictionary = NSMutableDictionary()
self.container[key.stringValue] = dictionary
self.codingPath.append(key)
defer { self.codingPath.removeLast() }
let container = _JSONKeyedEncodingContainer<NestedKey>(referencing: self.encoder, codingPath: self.codingPath, wrapping: dictionary)
return KeyedEncodingContainer(container)
}
public mutating func nestedUnkeyedContainer(forKey key: Key) -> UnkeyedEncodingContainer {
let array = NSMutableArray()
self.container[key.stringValue] = array
self.codingPath.append(key)
defer { self.codingPath.removeLast() }
return _JSONUnkeyedEncodingContainer(referencing: self.encoder, codingPath: self.codingPath, wrapping: array)
}
public mutating func superEncoder() -> Encoder {
return _JSONReferencingEncoder(referencing: self.encoder, at: _JSONKey.super, wrapping: self.container)
}
public mutating func superEncoder(forKey key: Key) -> Encoder {
return _JSONReferencingEncoder(referencing: self.encoder, at: key, wrapping: self.container)
}
}
fileprivate struct _JSONUnkeyedEncodingContainer : UnkeyedEncodingContainer {
// MARK: Properties
/// A reference to the encoder we're writing to.
private let encoder: _JSONEncoder
/// A reference to the container we're writing to.
private let container: NSMutableArray
/// The path of coding keys taken to get to this point in encoding.
private(set) public var codingPath: [CodingKey]
/// The number of elements encoded into the container.
public var count: Int {
return self.container.count
}
// MARK: - Initialization
/// Initializes `self` with the given references.
fileprivate init(referencing encoder: _JSONEncoder, codingPath: [CodingKey], wrapping container: NSMutableArray) {
self.encoder = encoder
self.codingPath = codingPath
self.container = container
}
// MARK: - UnkeyedEncodingContainer Methods
public mutating func encodeNil() throws { self.container.add(NSNull()) }
public mutating func encode(_ value: Bool) throws { self.container.add(self.encoder.box(value)) }
public mutating func encode(_ value: Int) throws { self.container.add(self.encoder.box(value)) }
public mutating func encode(_ value: Int8) throws { self.container.add(self.encoder.box(value)) }
public mutating func encode(_ value: Int16) throws { self.container.add(self.encoder.box(value)) }
public mutating func encode(_ value: Int32) throws { self.container.add(self.encoder.box(value)) }
public mutating func encode(_ value: Int64) throws { self.container.add(self.encoder.box(value)) }
public mutating func encode(_ value: UInt) throws { self.container.add(self.encoder.box(value)) }
public mutating func encode(_ value: UInt8) throws { self.container.add(self.encoder.box(value)) }
public mutating func encode(_ value: UInt16) throws { self.container.add(self.encoder.box(value)) }
public mutating func encode(_ value: UInt32) throws { self.container.add(self.encoder.box(value)) }
public mutating func encode(_ value: UInt64) throws { self.container.add(self.encoder.box(value)) }
public mutating func encode(_ value: String) throws { self.container.add(self.encoder.box(value)) }
public mutating func encode(_ value: Float) throws {
// Since the float may be invalid and throw, the coding path needs to contain this key.
self.encoder.codingPath.append(_JSONKey(index: self.count))
defer { self.encoder.codingPath.removeLast() }
self.container.add(try self.encoder.box(value))
}
public mutating func encode(_ value: Double) throws {
// Since the double may be invalid and throw, the coding path needs to contain this key.
self.encoder.codingPath.append(_JSONKey(index: self.count))
defer { self.encoder.codingPath.removeLast() }
self.container.add(try self.encoder.box(value))
}
public mutating func encode<T : Encodable>(_ value: T) throws {
self.encoder.codingPath.append(_JSONKey(index: self.count))
defer { self.encoder.codingPath.removeLast() }
self.container.add(try self.encoder.box(value))
}
public mutating func nestedContainer<NestedKey>(keyedBy keyType: NestedKey.Type) -> KeyedEncodingContainer<NestedKey> {
self.codingPath.append(_JSONKey(index: self.count))
defer { self.codingPath.removeLast() }
let dictionary = NSMutableDictionary()
self.container.add(dictionary)
let container = _JSONKeyedEncodingContainer<NestedKey>(referencing: self.encoder, codingPath: self.codingPath, wrapping: dictionary)
return KeyedEncodingContainer(container)
}
public mutating func nestedUnkeyedContainer() -> UnkeyedEncodingContainer {
self.codingPath.append(_JSONKey(index: self.count))
defer { self.codingPath.removeLast() }
let array = NSMutableArray()
self.container.add(array)
return _JSONUnkeyedEncodingContainer(referencing: self.encoder, codingPath: self.codingPath, wrapping: array)
}
public mutating func superEncoder() -> Encoder {
return _JSONReferencingEncoder(referencing: self.encoder, at: self.container.count, wrapping: self.container)
}
}
extension _JSONEncoder : SingleValueEncodingContainer {
// MARK: - SingleValueEncodingContainer Methods
fileprivate func assertCanEncodeNewValue() {
precondition(self.canEncodeNewValue, "Attempt to encode value through single value container when previously value already encoded.")
}
public func encodeNil() throws {
assertCanEncodeNewValue()
self.storage.push(container: NSNull())
}
public func encode(_ value: Bool) throws {
assertCanEncodeNewValue()
self.storage.push(container: box(value))
}
public func encode(_ value: Int) throws {
assertCanEncodeNewValue()
self.storage.push(container: box(value))
}
public func encode(_ value: Int8) throws {
assertCanEncodeNewValue()
self.storage.push(container: box(value))
}
public func encode(_ value: Int16) throws {
assertCanEncodeNewValue()
self.storage.push(container: box(value))
}
public func encode(_ value: Int32) throws {
assertCanEncodeNewValue()
self.storage.push(container: box(value))
}
public func encode(_ value: Int64) throws {
assertCanEncodeNewValue()
self.storage.push(container: box(value))
}
public func encode(_ value: UInt) throws {
assertCanEncodeNewValue()
self.storage.push(container: box(value))
}
public func encode(_ value: UInt8) throws {
assertCanEncodeNewValue()
self.storage.push(container: box(value))
}
public func encode(_ value: UInt16) throws {
assertCanEncodeNewValue()
self.storage.push(container: box(value))
}
public func encode(_ value: UInt32) throws {
assertCanEncodeNewValue()
self.storage.push(container: box(value))
}
public func encode(_ value: UInt64) throws {
assertCanEncodeNewValue()
self.storage.push(container: box(value))
}
public func encode(_ value: String) throws {
assertCanEncodeNewValue()
self.storage.push(container: box(value))
}
public func encode(_ value: Float) throws {
assertCanEncodeNewValue()
try self.storage.push(container: box(value))
}
public func encode(_ value: Double) throws {
assertCanEncodeNewValue()
try self.storage.push(container: box(value))
}
public func encode<T : Encodable>(_ value: T) throws {
assertCanEncodeNewValue()
try self.storage.push(container: box(value))
}
}
// MARK: - Concrete Value Representations
extension _JSONEncoder {
/// Returns the given value boxed in a container appropriate for pushing onto the container stack.
fileprivate func box(_ value: Bool) -> NSObject { return NSNumber(value: value) }
fileprivate func box(_ value: Int) -> NSObject { return NSNumber(value: value) }
fileprivate func box(_ value: Int8) -> NSObject { return NSNumber(value: value) }
fileprivate func box(_ value: Int16) -> NSObject { return NSNumber(value: value) }
fileprivate func box(_ value: Int32) -> NSObject { return NSNumber(value: value) }
fileprivate func box(_ value: Int64) -> NSObject { return NSNumber(value: value) }
fileprivate func box(_ value: UInt) -> NSObject { return NSNumber(value: value) }
fileprivate func box(_ value: UInt8) -> NSObject { return NSNumber(value: value) }
fileprivate func box(_ value: UInt16) -> NSObject { return NSNumber(value: value) }
fileprivate func box(_ value: UInt32) -> NSObject { return NSNumber(value: value) }
fileprivate func box(_ value: UInt64) -> NSObject { return NSNumber(value: value) }
fileprivate func box(_ value: String) -> NSObject { return NSString(string: value) }
fileprivate func box(_ float: Float) throws -> NSObject {
guard !float.isInfinite && !float.isNaN else {
guard case let .convertToString(positiveInfinity: posInfString,
negativeInfinity: negInfString,
nan: nanString) = self.options.nonConformingFloatEncodingStrategy else {
throw EncodingError._invalidFloatingPointValue(float, at: codingPath)
}
if float == Float.infinity {
return NSString(string: posInfString)
} else if float == -Float.infinity {
return NSString(string: negInfString)
} else {
return NSString(string: nanString)
}
}
return NSNumber(value: float)
}
fileprivate func box(_ double: Double) throws -> NSObject {
guard !double.isInfinite && !double.isNaN else {
guard case let .convertToString(positiveInfinity: posInfString,
negativeInfinity: negInfString,
nan: nanString) = self.options.nonConformingFloatEncodingStrategy else {
throw EncodingError._invalidFloatingPointValue(double, at: codingPath)
}
if double == Double.infinity {
return NSString(string: posInfString)
} else if double == -Double.infinity {
return NSString(string: negInfString)
} else {
return NSString(string: nanString)
}
}
return NSNumber(value: double)
}
fileprivate func box(_ date: Date) throws -> NSObject {
switch self.options.dateEncodingStrategy {
case .deferredToDate:
// Must be called with a surrounding with(pushedKey:) call.
try date.encode(to: self)
return self.storage.popContainer()
case .secondsSince1970:
return NSNumber(value: date.timeIntervalSince1970)
case .millisecondsSince1970:
return NSNumber(value: 1000.0 * date.timeIntervalSince1970)
case .iso8601:
if #available(OSX 10.12, iOS 10.0, watchOS 3.0, tvOS 10.0, *) {
return NSString(string: _iso8601Formatter.string(from: date))
} else {
fatalError("ISO8601DateFormatter is unavailable on this platform.")
}
case .formatted(let formatter):
return NSString(string: formatter.string(from: date))
case .custom(let closure):
let depth = self.storage.count
try closure(date, self)
guard self.storage.count > depth else {
// The closure didn't encode anything. Return the default keyed container.
return NSDictionary()
}
// We can pop because the closure encoded something.
return self.storage.popContainer()
}
}
fileprivate func box(_ data: Data) throws -> NSObject {
switch self.options.dataEncodingStrategy {
case .deferredToData:
// Must be called with a surrounding with(pushedKey:) call.
try data.encode(to: self)
return self.storage.popContainer()
case .base64:
return NSString(string: data.base64EncodedString())
case .custom(let closure):
let depth = self.storage.count
try closure(data, self)
guard self.storage.count > depth else {
// The closure didn't encode anything. Return the default keyed container.
return NSDictionary()
}
// We can pop because the closure encoded something.
return self.storage.popContainer()
}
}
fileprivate func box<T : Encodable>(_ value: T) throws -> NSObject {
if T.self == Date.self {
// Respect Date encoding strategy
return try self.box((value as! Date))
} else if T.self == Data.self {
// Respect Data encoding strategy
return try self.box((value as! Data))
} else if T.self == URL.self {
// Encode URLs as single strings.
return self.box((value as! URL).absoluteString)
} else if T.self == Decimal.self {
// JSONSerialization can natively handle NSDecimalNumber.
return (value as! Decimal) as NSDecimalNumber
}
// The value should request a container from the _JSONEncoder.
let topContainer = self.storage.containers.last
try value.encode(to: self)
// The top container should be a new container.
guard self.storage.containers.last! !== topContainer else {
// If the value didn't request a container at all, encode the default container instead.
return NSDictionary()
}
return self.storage.popContainer()
}
}
// MARK: - _JSONReferencingEncoder
/// _JSONReferencingEncoder is a special subclass of _JSONEncoder which has its own storage, but references the contents of a different encoder.
/// It's used in superEncoder(), which returns a new encoder for encoding a superclass -- the lifetime of the encoder should not escape the scope it's created in, but it doesn't necessarily know when it's done being used (to write to the original container).
fileprivate class _JSONReferencingEncoder : _JSONEncoder {
// MARK: Reference types.
/// The type of container we're referencing.
private enum Reference {
/// Referencing a specific index in an array container.
case array(NSMutableArray, Int)
/// Referencing a specific key in a dictionary container.
case dictionary(NSMutableDictionary, String)
}
// MARK: - Properties
/// The encoder we're referencing.
fileprivate let encoder: _JSONEncoder
/// The container reference itself.
private let reference: Reference
// MARK: - Initialization
/// Initializes `self` by referencing the given array container in the given encoder.
fileprivate init(referencing encoder: _JSONEncoder, at index: Int, wrapping array: NSMutableArray) {
self.encoder = encoder
self.reference = .array(array, index)
super.init(options: encoder.options, codingPath: encoder.codingPath)
self.codingPath.append(_JSONKey(index: index))
}
/// Initializes `self` by referencing the given dictionary container in the given encoder.
fileprivate init(referencing encoder: _JSONEncoder, at key: CodingKey, wrapping dictionary: NSMutableDictionary) {
self.encoder = encoder
self.reference = .dictionary(dictionary, key.stringValue)
super.init(options: encoder.options, codingPath: encoder.codingPath)
self.codingPath.append(key)
}
// MARK: - Coding Path Operations
fileprivate override var canEncodeNewValue: Bool {
// With a regular encoder, the storage and coding path grow together.
// A referencing encoder, however, inherits its parents coding path, as well as the key it was created for.
// We have to take this into account.
return self.storage.count == self.codingPath.count - self.encoder.codingPath.count - 1
}
// MARK: - Deinitialization
// Finalizes `self` by writing the contents of our storage to the referenced encoder's storage.
deinit {
let value: Any
switch self.storage.count {
case 0: value = NSDictionary()
case 1: value = self.storage.popContainer()
default: fatalError("Referencing encoder deallocated with multiple containers on stack.")
}
switch self.reference {
case .array(let array, let index):
array.insert(value, at: index)
case .dictionary(let dictionary, let key):
dictionary[NSString(string: key)] = value
}
}
}
//===----------------------------------------------------------------------===//
// JSON Decoder
//===----------------------------------------------------------------------===//
/// `JSONDecoder` facilitates the decoding of JSON into semantic `Decodable` types.
open class JSONDecoder {
// MARK: Options
/// The strategy to use for decoding `Date` values.
public enum DateDecodingStrategy {
/// Defer to `Date` for decoding. This is the default strategy.
case deferredToDate
/// Decode the `Date` as a UNIX timestamp from a JSON number.
case secondsSince1970
/// Decode the `Date` as UNIX millisecond timestamp from a JSON number.
case millisecondsSince1970
/// Decode the `Date` as an ISO-8601-formatted string (in RFC 3339 format).
@available(OSX 10.12, iOS 10.0, watchOS 3.0, tvOS 10.0, *)
case iso8601
/// Decode the `Date` as a string parsed by the given formatter.
case formatted(DateFormatter)
/// Decode the `Date` as a custom value decoded by the given closure.
case custom((_ decoder: Decoder) throws -> Date)
}
/// The strategy to use for decoding `Data` values.
public enum DataDecodingStrategy {
/// Defer to `Data` for decoding.
case deferredToData
/// Decode the `Data` from a Base64-encoded string. This is the default strategy.
case base64
/// Decode the `Data` as a custom value decoded by the given closure.
case custom((_ decoder: Decoder) throws -> Data)
}
/// The strategy to use for non-JSON-conforming floating-point values (IEEE 754 infinity and NaN).
public enum NonConformingFloatDecodingStrategy {
/// Throw upon encountering non-conforming values. This is the default strategy.
case `throw`
/// Decode the values from the given representation strings.
case convertFromString(positiveInfinity: String, negativeInfinity: String, nan: String)
}
/// The strategy to use in decoding dates. Defaults to `.deferredToDate`.
open var dateDecodingStrategy: DateDecodingStrategy = .deferredToDate
/// The strategy to use in decoding binary data. Defaults to `.base64`.
open var dataDecodingStrategy: DataDecodingStrategy = .base64
/// The strategy to use in decoding non-conforming numbers. Defaults to `.throw`.
open var nonConformingFloatDecodingStrategy: NonConformingFloatDecodingStrategy = .throw
/// Contextual user-provided information for use during decoding.
open var userInfo: [CodingUserInfoKey : Any] = [:]
/// Options set on the top-level encoder to pass down the decoding hierarchy.
fileprivate struct _Options {
let dateDecodingStrategy: DateDecodingStrategy
let dataDecodingStrategy: DataDecodingStrategy
let nonConformingFloatDecodingStrategy: NonConformingFloatDecodingStrategy
let userInfo: [CodingUserInfoKey : Any]
}
/// The options set on the top-level decoder.
fileprivate var options: _Options {
return _Options(dateDecodingStrategy: dateDecodingStrategy,
dataDecodingStrategy: dataDecodingStrategy,
nonConformingFloatDecodingStrategy: nonConformingFloatDecodingStrategy,
userInfo: userInfo)
}
// MARK: - Constructing a JSON Decoder
/// Initializes `self` with default strategies.
public init() {}
// MARK: - Decoding Values
/// Decodes a top-level value of the given type from the given JSON representation.
///
/// - parameter type: The type of the value to decode.
/// - parameter data: The data to decode from.
/// - returns: A value of the requested type.
/// - throws: `DecodingError.dataCorrupted` if values requested from the payload are corrupted, or if the given data is not valid JSON.
/// - throws: An error if any value throws an error during decoding.
open func decode<T : Decodable>(_ type: T.Type, from data: Data) throws -> T {
let topLevel: Any
do {
topLevel = try JSONSerialization.jsonObject(with: data)
} catch {
throw DecodingError.dataCorrupted(DecodingError.Context(codingPath: [], debugDescription: "The given data was not valid JSON.", underlyingError: error))
}
let decoder = _JSONDecoder(referencing: topLevel, options: self.options)
return try T(from: decoder)
}
}
// MARK: - _JSONDecoder
fileprivate class _JSONDecoder : Decoder {
// MARK: Properties
/// The decoder's storage.
fileprivate var storage: _JSONDecodingStorage
/// Options set on the top-level decoder.
fileprivate let options: JSONDecoder._Options
/// The path to the current point in encoding.
fileprivate(set) public var codingPath: [CodingKey]
/// Contextual user-provided information for use during encoding.
public var userInfo: [CodingUserInfoKey : Any] {
return self.options.userInfo
}
// MARK: - Initialization
/// Initializes `self` with the given top-level container and options.
fileprivate init(referencing container: Any, at codingPath: [CodingKey] = [], options: JSONDecoder._Options) {
self.storage = _JSONDecodingStorage()
self.storage.push(container: container)
self.codingPath = codingPath
self.options = options
}
// MARK: - Decoder Methods
public func container<Key>(keyedBy type: Key.Type) throws -> KeyedDecodingContainer<Key> {
guard !(self.storage.topContainer is NSNull) else {
throw DecodingError.valueNotFound(KeyedDecodingContainer<Key>.self,
DecodingError.Context(codingPath: self.codingPath,
debugDescription: "Cannot get keyed decoding container -- found null value instead."))
}
guard let topContainer = self.storage.topContainer as? [String : Any] else {
throw DecodingError._typeMismatch(at: self.codingPath, expectation: [String : Any].self, reality: self.storage.topContainer)
}
let container = _JSONKeyedDecodingContainer<Key>(referencing: self, wrapping: topContainer)
return KeyedDecodingContainer(container)
}
public func unkeyedContainer() throws -> UnkeyedDecodingContainer {
guard !(self.storage.topContainer is NSNull) else {
throw DecodingError.valueNotFound(UnkeyedDecodingContainer.self,
DecodingError.Context(codingPath: self.codingPath,
debugDescription: "Cannot get unkeyed decoding container -- found null value instead."))
}
guard let topContainer = self.storage.topContainer as? [Any] else {
throw DecodingError._typeMismatch(at: self.codingPath, expectation: [Any].self, reality: self.storage.topContainer)
}
return _JSONUnkeyedDecodingContainer(referencing: self, wrapping: topContainer)
}
public func singleValueContainer() throws -> SingleValueDecodingContainer {
return self
}
}
// MARK: - Decoding Storage
fileprivate struct _JSONDecodingStorage {
// MARK: Properties
/// The container stack.
/// Elements may be any one of the JSON types (NSNull, NSNumber, String, Array, [String : Any]).
private(set) fileprivate var containers: [Any] = []
// MARK: - Initialization
/// Initializes `self` with no containers.
fileprivate init() {}
// MARK: - Modifying the Stack
fileprivate var count: Int {
return self.containers.count
}
fileprivate var topContainer: Any {
precondition(self.containers.count > 0, "Empty container stack.")
return self.containers.last!
}
fileprivate mutating func push(container: Any) {
self.containers.append(container)
}
fileprivate mutating func popContainer() {
precondition(self.containers.count > 0, "Empty container stack.")
self.containers.removeLast()
}
}
// MARK: Decoding Containers
fileprivate struct _JSONKeyedDecodingContainer<K : CodingKey> : KeyedDecodingContainerProtocol {
typealias Key = K
// MARK: Properties
/// A reference to the decoder we're reading from.
private let decoder: _JSONDecoder
/// A reference to the container we're reading from.
private let container: [String : Any]
/// The path of coding keys taken to get to this point in decoding.
private(set) public var codingPath: [CodingKey]
// MARK: - Initialization
/// Initializes `self` by referencing the given decoder and container.
fileprivate init(referencing decoder: _JSONDecoder, wrapping container: [String : Any]) {
self.decoder = decoder
self.container = container
self.codingPath = decoder.codingPath
}
// MARK: - KeyedDecodingContainerProtocol Methods
public var allKeys: [Key] {
return self.container.keys.flatMap { Key(stringValue: $0) }
}
public func contains(_ key: Key) -> Bool {
return self.container[key.stringValue] != nil
}
public func decodeNil(forKey key: Key) throws -> Bool {
guard let entry = self.container[key.stringValue] else {
throw DecodingError.keyNotFound(key, DecodingError.Context(codingPath: self.decoder.codingPath, debugDescription: "No value associated with key \(key) (\"\(key.stringValue)\")."))
}
return entry is NSNull
}
public func decode(_ type: Bool.Type, forKey key: Key) throws -> Bool {
guard let entry = self.container[key.stringValue] else {
throw DecodingError.keyNotFound(key, DecodingError.Context(codingPath: self.decoder.codingPath, debugDescription: "No value associated with key \(key) (\"\(key.stringValue)\")."))
}
self.decoder.codingPath.append(key)
defer { self.decoder.codingPath.removeLast() }
guard let value = try self.decoder.unbox(entry, as: Bool.self) else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath, debugDescription: "Expected \(type) value but found null instead."))
}
return value
}
public func decode(_ type: Int.Type, forKey key: Key) throws -> Int {
guard let entry = self.container[key.stringValue] else {
throw DecodingError.keyNotFound(key, DecodingError.Context(codingPath: self.decoder.codingPath, debugDescription: "No value associated with key \(key) (\"\(key.stringValue)\")."))
}
self.decoder.codingPath.append(key)
defer { self.decoder.codingPath.removeLast() }
guard let value = try self.decoder.unbox(entry, as: Int.self) else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath, debugDescription: "Expected \(type) value but found null instead."))
}
return value
}
public func decode(_ type: Int8.Type, forKey key: Key) throws -> Int8 {
guard let entry = self.container[key.stringValue] else {
throw DecodingError.keyNotFound(key, DecodingError.Context(codingPath: self.decoder.codingPath, debugDescription: "No value associated with key \(key) (\"\(key.stringValue)\")."))
}
self.decoder.codingPath.append(key)
defer { self.decoder.codingPath.removeLast() }
guard let value = try self.decoder.unbox(entry, as: Int8.self) else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath, debugDescription: "Expected \(type) value but found null instead."))
}
return value
}
public func decode(_ type: Int16.Type, forKey key: Key) throws -> Int16 {
guard let entry = self.container[key.stringValue] else {
throw DecodingError.keyNotFound(key, DecodingError.Context(codingPath: self.decoder.codingPath, debugDescription: "No value associated with key \(key) (\"\(key.stringValue)\")."))
}
self.decoder.codingPath.append(key)
defer { self.decoder.codingPath.removeLast() }
guard let value = try self.decoder.unbox(entry, as: Int16.self) else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath, debugDescription: "Expected \(type) value but found null instead."))
}
return value
}
public func decode(_ type: Int32.Type, forKey key: Key) throws -> Int32 {
guard let entry = self.container[key.stringValue] else {
throw DecodingError.keyNotFound(key, DecodingError.Context(codingPath: self.decoder.codingPath, debugDescription: "No value associated with key \(key) (\"\(key.stringValue)\")."))
}
self.decoder.codingPath.append(key)
defer { self.decoder.codingPath.removeLast() }
guard let value = try self.decoder.unbox(entry, as: Int32.self) else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath, debugDescription: "Expected \(type) value but found null instead."))
}
return value
}
public func decode(_ type: Int64.Type, forKey key: Key) throws -> Int64 {
guard let entry = self.container[key.stringValue] else {
throw DecodingError.keyNotFound(key, DecodingError.Context(codingPath: self.decoder.codingPath, debugDescription: "No value associated with key \(key) (\"\(key.stringValue)\")."))
}
self.decoder.codingPath.append(key)
defer { self.decoder.codingPath.removeLast() }
guard let value = try self.decoder.unbox(entry, as: Int64.self) else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath, debugDescription: "Expected \(type) value but found null instead."))
}
return value
}
public func decode(_ type: UInt.Type, forKey key: Key) throws -> UInt {
guard let entry = self.container[key.stringValue] else {
throw DecodingError.keyNotFound(key, DecodingError.Context(codingPath: self.decoder.codingPath, debugDescription: "No value associated with key \(key) (\"\(key.stringValue)\")."))
}
self.decoder.codingPath.append(key)
defer { self.decoder.codingPath.removeLast() }
guard let value = try self.decoder.unbox(entry, as: UInt.self) else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath, debugDescription: "Expected \(type) value but found null instead."))
}
return value
}
public func decode(_ type: UInt8.Type, forKey key: Key) throws -> UInt8 {
guard let entry = self.container[key.stringValue] else {
throw DecodingError.keyNotFound(key, DecodingError.Context(codingPath: self.decoder.codingPath, debugDescription: "No value associated with key \(key) (\"\(key.stringValue)\")."))
}
self.decoder.codingPath.append(key)
defer { self.decoder.codingPath.removeLast() }
guard let value = try self.decoder.unbox(entry, as: UInt8.self) else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath, debugDescription: "Expected \(type) value but found null instead."))
}
return value
}
public func decode(_ type: UInt16.Type, forKey key: Key) throws -> UInt16 {
guard let entry = self.container[key.stringValue] else {
throw DecodingError.keyNotFound(key, DecodingError.Context(codingPath: self.decoder.codingPath, debugDescription: "No value associated with key \(key) (\"\(key.stringValue)\")."))
}
self.decoder.codingPath.append(key)
defer { self.decoder.codingPath.removeLast() }
guard let value = try self.decoder.unbox(entry, as: UInt16.self) else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath, debugDescription: "Expected \(type) value but found null instead."))
}
return value
}
public func decode(_ type: UInt32.Type, forKey key: Key) throws -> UInt32 {
guard let entry = self.container[key.stringValue] else {
throw DecodingError.keyNotFound(key, DecodingError.Context(codingPath: self.decoder.codingPath, debugDescription: "No value associated with key \(key) (\"\(key.stringValue)\")."))
}
self.decoder.codingPath.append(key)
defer { self.decoder.codingPath.removeLast() }
guard let value = try self.decoder.unbox(entry, as: UInt32.self) else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath, debugDescription: "Expected \(type) value but found null instead."))
}
return value
}
public func decode(_ type: UInt64.Type, forKey key: Key) throws -> UInt64 {
guard let entry = self.container[key.stringValue] else {
throw DecodingError.keyNotFound(key, DecodingError.Context(codingPath: self.decoder.codingPath, debugDescription: "No value associated with key \(key) (\"\(key.stringValue)\")."))
}
self.decoder.codingPath.append(key)
defer { self.decoder.codingPath.removeLast() }
guard let value = try self.decoder.unbox(entry, as: UInt64.self) else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath, debugDescription: "Expected \(type) value but found null instead."))
}
return value
}
public func decode(_ type: Float.Type, forKey key: Key) throws -> Float {
guard let entry = self.container[key.stringValue] else {
throw DecodingError.keyNotFound(key, DecodingError.Context(codingPath: self.decoder.codingPath, debugDescription: "No value associated with key \(key) (\"\(key.stringValue)\")."))
}
self.decoder.codingPath.append(key)
defer { self.decoder.codingPath.removeLast() }
guard let value = try self.decoder.unbox(entry, as: Float.self) else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath, debugDescription: "Expected \(type) value but found null instead."))
}
return value
}
public func decode(_ type: Double.Type, forKey key: Key) throws -> Double {
guard let entry = self.container[key.stringValue] else {
throw DecodingError.keyNotFound(key, DecodingError.Context(codingPath: self.decoder.codingPath, debugDescription: "No value associated with key \(key) (\"\(key.stringValue)\")."))
}
self.decoder.codingPath.append(key)
defer { self.decoder.codingPath.removeLast() }
guard let value = try self.decoder.unbox(entry, as: Double.self) else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath, debugDescription: "Expected \(type) value but found null instead."))
}
return value
}
public func decode(_ type: String.Type, forKey key: Key) throws -> String {
guard let entry = self.container[key.stringValue] else {
throw DecodingError.keyNotFound(key, DecodingError.Context(codingPath: self.decoder.codingPath, debugDescription: "No value associated with key \(key) (\"\(key.stringValue)\")."))
}
self.decoder.codingPath.append(key)
defer { self.decoder.codingPath.removeLast() }
guard let value = try self.decoder.unbox(entry, as: String.self) else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath, debugDescription: "Expected \(type) value but found null instead."))
}
return value
}
public func decode<T : Decodable>(_ type: T.Type, forKey key: Key) throws -> T {
guard let entry = self.container[key.stringValue] else {
throw DecodingError.keyNotFound(key, DecodingError.Context(codingPath: self.decoder.codingPath, debugDescription: "No value associated with key \(key) (\"\(key.stringValue)\")."))
}
self.decoder.codingPath.append(key)
defer { self.decoder.codingPath.removeLast() }
guard let value = try self.decoder.unbox(entry, as: T.self) else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath, debugDescription: "Expected \(type) value but found null instead."))
}
return value
}
public func nestedContainer<NestedKey>(keyedBy type: NestedKey.Type, forKey key: Key) throws -> KeyedDecodingContainer<NestedKey> {
self.decoder.codingPath.append(key)
defer { self.decoder.codingPath.removeLast() }
guard let value = self.container[key.stringValue] else {
throw DecodingError.keyNotFound(key,
DecodingError.Context(codingPath: self.codingPath,
debugDescription: "Cannot get \(KeyedDecodingContainer<NestedKey>.self) -- no value found for key \"\(key.stringValue)\""))
}
guard let dictionary = value as? [String : Any] else {
throw DecodingError._typeMismatch(at: self.codingPath, expectation: [String : Any].self, reality: value)
}
let container = _JSONKeyedDecodingContainer<NestedKey>(referencing: self.decoder, wrapping: dictionary)
return KeyedDecodingContainer(container)
}
public func nestedUnkeyedContainer(forKey key: Key) throws -> UnkeyedDecodingContainer {
self.decoder.codingPath.append(key)
defer { self.decoder.codingPath.removeLast() }
guard let value = self.container[key.stringValue] else {
throw DecodingError.keyNotFound(key,
DecodingError.Context(codingPath: self.codingPath,
debugDescription: "Cannot get UnkeyedDecodingContainer -- no value found for key \"\(key.stringValue)\""))
}
guard let array = value as? [Any] else {
throw DecodingError._typeMismatch(at: self.codingPath, expectation: [Any].self, reality: value)
}
return _JSONUnkeyedDecodingContainer(referencing: self.decoder, wrapping: array)
}
private func _superDecoder(forKey key: CodingKey) throws -> Decoder {
self.decoder.codingPath.append(key)
defer { self.decoder.codingPath.removeLast() }
let value: Any = self.container[key.stringValue] ?? NSNull()
return _JSONDecoder(referencing: value, at: self.decoder.codingPath, options: self.decoder.options)
}
public func superDecoder() throws -> Decoder {
return try _superDecoder(forKey: _JSONKey.super)
}
public func superDecoder(forKey key: Key) throws -> Decoder {
return try _superDecoder(forKey: key)
}
}
fileprivate struct _JSONUnkeyedDecodingContainer : UnkeyedDecodingContainer {
// MARK: Properties
/// A reference to the decoder we're reading from.
private let decoder: _JSONDecoder
/// A reference to the container we're reading from.
private let container: [Any]
/// The path of coding keys taken to get to this point in decoding.
private(set) public var codingPath: [CodingKey]
/// The index of the element we're about to decode.
private(set) public var currentIndex: Int
// MARK: - Initialization
/// Initializes `self` by referencing the given decoder and container.
fileprivate init(referencing decoder: _JSONDecoder, wrapping container: [Any]) {
self.decoder = decoder
self.container = container
self.codingPath = decoder.codingPath
self.currentIndex = 0
}
// MARK: - UnkeyedDecodingContainer Methods
public var count: Int? {
return self.container.count
}
public var isAtEnd: Bool {
return self.currentIndex >= self.count!
}
public mutating func decodeNil() throws -> Bool {
guard !self.isAtEnd else {
throw DecodingError.valueNotFound(Any?.self, DecodingError.Context(codingPath: self.decoder.codingPath + [_JSONKey(index: self.currentIndex)], debugDescription: "Unkeyed container is at end."))
}
if self.container[self.currentIndex] is NSNull {
self.currentIndex += 1
return true
} else {
return false
}
}
public mutating func decode(_ type: Bool.Type) throws -> Bool {
guard !self.isAtEnd else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath + [_JSONKey(index: self.currentIndex)], debugDescription: "Unkeyed container is at end."))
}
self.decoder.codingPath.append(_JSONKey(index: self.currentIndex))
defer { self.decoder.codingPath.removeLast() }
guard let decoded = try self.decoder.unbox(self.container[self.currentIndex], as: Bool.self) else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath + [_JSONKey(index: self.currentIndex)], debugDescription: "Expected \(type) but found null instead."))
}
self.currentIndex += 1
return decoded
}
public mutating func decode(_ type: Int.Type) throws -> Int {
guard !self.isAtEnd else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath + [_JSONKey(index: self.currentIndex)], debugDescription: "Unkeyed container is at end."))
}
self.decoder.codingPath.append(_JSONKey(index: self.currentIndex))
defer { self.decoder.codingPath.removeLast() }
guard let decoded = try self.decoder.unbox(self.container[self.currentIndex], as: Int.self) else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath + [_JSONKey(index: self.currentIndex)], debugDescription: "Expected \(type) but found null instead."))
}
self.currentIndex += 1
return decoded
}
public mutating func decode(_ type: Int8.Type) throws -> Int8 {
guard !self.isAtEnd else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath + [_JSONKey(index: self.currentIndex)], debugDescription: "Unkeyed container is at end."))
}
self.decoder.codingPath.append(_JSONKey(index: self.currentIndex))
defer { self.decoder.codingPath.removeLast() }
guard let decoded = try self.decoder.unbox(self.container[self.currentIndex], as: Int8.self) else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath + [_JSONKey(index: self.currentIndex)], debugDescription: "Expected \(type) but found null instead."))
}
self.currentIndex += 1
return decoded
}
public mutating func decode(_ type: Int16.Type) throws -> Int16 {
guard !self.isAtEnd else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath + [_JSONKey(index: self.currentIndex)], debugDescription: "Unkeyed container is at end."))
}
self.decoder.codingPath.append(_JSONKey(index: self.currentIndex))
defer { self.decoder.codingPath.removeLast() }
guard let decoded = try self.decoder.unbox(self.container[self.currentIndex], as: Int16.self) else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath + [_JSONKey(index: self.currentIndex)], debugDescription: "Expected \(type) but found null instead."))
}
self.currentIndex += 1
return decoded
}
public mutating func decode(_ type: Int32.Type) throws -> Int32 {
guard !self.isAtEnd else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath + [_JSONKey(index: self.currentIndex)], debugDescription: "Unkeyed container is at end."))
}
self.decoder.codingPath.append(_JSONKey(index: self.currentIndex))
defer { self.decoder.codingPath.removeLast() }
guard let decoded = try self.decoder.unbox(self.container[self.currentIndex], as: Int32.self) else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath + [_JSONKey(index: self.currentIndex)], debugDescription: "Expected \(type) but found null instead."))
}
self.currentIndex += 1
return decoded
}
public mutating func decode(_ type: Int64.Type) throws -> Int64 {
guard !self.isAtEnd else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath + [_JSONKey(index: self.currentIndex)], debugDescription: "Unkeyed container is at end."))
}
self.decoder.codingPath.append(_JSONKey(index: self.currentIndex))
defer { self.decoder.codingPath.removeLast() }
guard let decoded = try self.decoder.unbox(self.container[self.currentIndex], as: Int64.self) else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath + [_JSONKey(index: self.currentIndex)], debugDescription: "Expected \(type) but found null instead."))
}
self.currentIndex += 1
return decoded
}
public mutating func decode(_ type: UInt.Type) throws -> UInt {
guard !self.isAtEnd else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath + [_JSONKey(index: self.currentIndex)], debugDescription: "Unkeyed container is at end."))
}
self.decoder.codingPath.append(_JSONKey(index: self.currentIndex))
defer { self.decoder.codingPath.removeLast() }
guard let decoded = try self.decoder.unbox(self.container[self.currentIndex], as: UInt.self) else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath + [_JSONKey(index: self.currentIndex)], debugDescription: "Expected \(type) but found null instead."))
}
self.currentIndex += 1
return decoded
}
public mutating func decode(_ type: UInt8.Type) throws -> UInt8 {
guard !self.isAtEnd else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath + [_JSONKey(index: self.currentIndex)], debugDescription: "Unkeyed container is at end."))
}
self.decoder.codingPath.append(_JSONKey(index: self.currentIndex))
defer { self.decoder.codingPath.removeLast() }
guard let decoded = try self.decoder.unbox(self.container[self.currentIndex], as: UInt8.self) else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath + [_JSONKey(index: self.currentIndex)], debugDescription: "Expected \(type) but found null instead."))
}
self.currentIndex += 1
return decoded
}
public mutating func decode(_ type: UInt16.Type) throws -> UInt16 {
guard !self.isAtEnd else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath + [_JSONKey(index: self.currentIndex)], debugDescription: "Unkeyed container is at end."))
}
self.decoder.codingPath.append(_JSONKey(index: self.currentIndex))
defer { self.decoder.codingPath.removeLast() }
guard let decoded = try self.decoder.unbox(self.container[self.currentIndex], as: UInt16.self) else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath + [_JSONKey(index: self.currentIndex)], debugDescription: "Expected \(type) but found null instead."))
}
self.currentIndex += 1
return decoded
}
public mutating func decode(_ type: UInt32.Type) throws -> UInt32 {
guard !self.isAtEnd else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath + [_JSONKey(index: self.currentIndex)], debugDescription: "Unkeyed container is at end."))
}
self.decoder.codingPath.append(_JSONKey(index: self.currentIndex))
defer { self.decoder.codingPath.removeLast() }
guard let decoded = try self.decoder.unbox(self.container[self.currentIndex], as: UInt32.self) else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath + [_JSONKey(index: self.currentIndex)], debugDescription: "Expected \(type) but found null instead."))
}
self.currentIndex += 1
return decoded
}
public mutating func decode(_ type: UInt64.Type) throws -> UInt64 {
guard !self.isAtEnd else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath + [_JSONKey(index: self.currentIndex)], debugDescription: "Unkeyed container is at end."))
}
self.decoder.codingPath.append(_JSONKey(index: self.currentIndex))
defer { self.decoder.codingPath.removeLast() }
guard let decoded = try self.decoder.unbox(self.container[self.currentIndex], as: UInt64.self) else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath + [_JSONKey(index: self.currentIndex)], debugDescription: "Expected \(type) but found null instead."))
}
self.currentIndex += 1
return decoded
}
public mutating func decode(_ type: Float.Type) throws -> Float {
guard !self.isAtEnd else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath + [_JSONKey(index: self.currentIndex)], debugDescription: "Unkeyed container is at end."))
}
self.decoder.codingPath.append(_JSONKey(index: self.currentIndex))
defer { self.decoder.codingPath.removeLast() }
guard let decoded = try self.decoder.unbox(self.container[self.currentIndex], as: Float.self) else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath + [_JSONKey(index: self.currentIndex)], debugDescription: "Expected \(type) but found null instead."))
}
self.currentIndex += 1
return decoded
}
public mutating func decode(_ type: Double.Type) throws -> Double {
guard !self.isAtEnd else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath + [_JSONKey(index: self.currentIndex)], debugDescription: "Unkeyed container is at end."))
}
self.decoder.codingPath.append(_JSONKey(index: self.currentIndex))
defer { self.decoder.codingPath.removeLast() }
guard let decoded = try self.decoder.unbox(self.container[self.currentIndex], as: Double.self) else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath + [_JSONKey(index: self.currentIndex)], debugDescription: "Expected \(type) but found null instead."))
}
self.currentIndex += 1
return decoded
}
public mutating func decode(_ type: String.Type) throws -> String {
guard !self.isAtEnd else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath + [_JSONKey(index: self.currentIndex)], debugDescription: "Unkeyed container is at end."))
}
self.decoder.codingPath.append(_JSONKey(index: self.currentIndex))
defer { self.decoder.codingPath.removeLast() }
guard let decoded = try self.decoder.unbox(self.container[self.currentIndex], as: String.self) else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath + [_JSONKey(index: self.currentIndex)], debugDescription: "Expected \(type) but found null instead."))
}
self.currentIndex += 1
return decoded
}
public mutating func decode<T : Decodable>(_ type: T.Type) throws -> T {
guard !self.isAtEnd else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath + [_JSONKey(index: self.currentIndex)], debugDescription: "Unkeyed container is at end."))
}
self.decoder.codingPath.append(_JSONKey(index: self.currentIndex))
defer { self.decoder.codingPath.removeLast() }
guard let decoded = try self.decoder.unbox(self.container[self.currentIndex], as: T.self) else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.decoder.codingPath + [_JSONKey(index: self.currentIndex)], debugDescription: "Expected \(type) but found null instead."))
}
self.currentIndex += 1
return decoded
}
public mutating func nestedContainer<NestedKey>(keyedBy type: NestedKey.Type) throws -> KeyedDecodingContainer<NestedKey> {
self.decoder.codingPath.append(_JSONKey(index: self.currentIndex))
defer { self.decoder.codingPath.removeLast() }
guard !self.isAtEnd else {
throw DecodingError.valueNotFound(KeyedDecodingContainer<NestedKey>.self,
DecodingError.Context(codingPath: self.codingPath,
debugDescription: "Cannot get nested keyed container -- unkeyed container is at end."))
}
let value = self.container[self.currentIndex]
guard !(value is NSNull) else {
throw DecodingError.valueNotFound(KeyedDecodingContainer<NestedKey>.self,
DecodingError.Context(codingPath: self.codingPath,
debugDescription: "Cannot get keyed decoding container -- found null value instead."))
}
guard let dictionary = value as? [String : Any] else {
throw DecodingError._typeMismatch(at: self.codingPath, expectation: [String : Any].self, reality: value)
}
self.currentIndex += 1
let container = _JSONKeyedDecodingContainer<NestedKey>(referencing: self.decoder, wrapping: dictionary)
return KeyedDecodingContainer(container)
}
public mutating func nestedUnkeyedContainer() throws -> UnkeyedDecodingContainer {
self.decoder.codingPath.append(_JSONKey(index: self.currentIndex))
defer { self.decoder.codingPath.removeLast() }
guard !self.isAtEnd else {
throw DecodingError.valueNotFound(UnkeyedDecodingContainer.self,
DecodingError.Context(codingPath: self.codingPath,
debugDescription: "Cannot get nested keyed container -- unkeyed container is at end."))
}
let value = self.container[self.currentIndex]
guard !(value is NSNull) else {
throw DecodingError.valueNotFound(UnkeyedDecodingContainer.self,
DecodingError.Context(codingPath: self.codingPath,
debugDescription: "Cannot get keyed decoding container -- found null value instead."))
}
guard let array = value as? [Any] else {
throw DecodingError._typeMismatch(at: self.codingPath, expectation: [Any].self, reality: value)
}
self.currentIndex += 1
return _JSONUnkeyedDecodingContainer(referencing: self.decoder, wrapping: array)
}
public mutating func superDecoder() throws -> Decoder {
self.decoder.codingPath.append(_JSONKey(index: self.currentIndex))
defer { self.decoder.codingPath.removeLast() }
guard !self.isAtEnd else {
throw DecodingError.valueNotFound(Decoder.self,
DecodingError.Context(codingPath: self.codingPath,
debugDescription: "Cannot get superDecoder() -- unkeyed container is at end."))
}
let value = self.container[self.currentIndex]
self.currentIndex += 1
return _JSONDecoder(referencing: value, at: self.decoder.codingPath, options: self.decoder.options)
}
}
extension _JSONDecoder : SingleValueDecodingContainer {
// MARK: SingleValueDecodingContainer Methods
private func expectNonNull<T>(_ type: T.Type) throws {
guard !self.decodeNil() else {
throw DecodingError.valueNotFound(type, DecodingError.Context(codingPath: self.codingPath, debugDescription: "Expected \(type) but found null value instead."))
}
}
public func decodeNil() -> Bool {
return self.storage.topContainer is NSNull
}
public func decode(_ type: Bool.Type) throws -> Bool {
try expectNonNull(Bool.self)
return try self.unbox(self.storage.topContainer, as: Bool.self)!
}
public func decode(_ type: Int.Type) throws -> Int {
try expectNonNull(Int.self)
return try self.unbox(self.storage.topContainer, as: Int.self)!
}
public func decode(_ type: Int8.Type) throws -> Int8 {
try expectNonNull(Int8.self)
return try self.unbox(self.storage.topContainer, as: Int8.self)!
}
public func decode(_ type: Int16.Type) throws -> Int16 {
try expectNonNull(Int16.self)
return try self.unbox(self.storage.topContainer, as: Int16.self)!
}
public func decode(_ type: Int32.Type) throws -> Int32 {
try expectNonNull(Int32.self)
return try self.unbox(self.storage.topContainer, as: Int32.self)!
}
public func decode(_ type: Int64.Type) throws -> Int64 {
try expectNonNull(Int64.self)
return try self.unbox(self.storage.topContainer, as: Int64.self)!
}
public func decode(_ type: UInt.Type) throws -> UInt {
try expectNonNull(UInt.self)
return try self.unbox(self.storage.topContainer, as: UInt.self)!
}
public func decode(_ type: UInt8.Type) throws -> UInt8 {
try expectNonNull(UInt8.self)
return try self.unbox(self.storage.topContainer, as: UInt8.self)!
}
public func decode(_ type: UInt16.Type) throws -> UInt16 {
try expectNonNull(UInt16.self)
return try self.unbox(self.storage.topContainer, as: UInt16.self)!
}
public func decode(_ type: UInt32.Type) throws -> UInt32 {
try expectNonNull(UInt32.self)
return try self.unbox(self.storage.topContainer, as: UInt32.self)!
}
public func decode(_ type: UInt64.Type) throws -> UInt64 {
try expectNonNull(UInt64.self)
return try self.unbox(self.storage.topContainer, as: UInt64.self)!
}
public func decode(_ type: Float.Type) throws -> Float {
try expectNonNull(Float.self)
return try self.unbox(self.storage.topContainer, as: Float.self)!
}
public func decode(_ type: Double.Type) throws -> Double {
try expectNonNull(Double.self)
return try self.unbox(self.storage.topContainer, as: Double.self)!
}
public func decode(_ type: String.Type) throws -> String {
try expectNonNull(String.self)
return try self.unbox(self.storage.topContainer, as: String.self)!
}
public func decode<T : Decodable>(_ type: T.Type) throws -> T {
try expectNonNull(T.self)
return try self.unbox(self.storage.topContainer, as: T.self)!
}
}
// MARK: - Concrete Value Representations
extension _JSONDecoder {
/// Returns the given value unboxed from a container.
fileprivate func unbox(_ value: Any, as type: Bool.Type) throws -> Bool? {
guard !(value is NSNull) else { return nil }
if let number = value as? NSNumber {
// TODO: Add a flag to coerce non-boolean numbers into Bools?
if number === kCFBooleanTrue as NSNumber {
return true
} else if number === kCFBooleanFalse as NSNumber {
return false
}
/* FIXME: If swift-corelibs-foundation doesn't change to use NSNumber, this code path will need to be included and tested:
} else if let bool = value as? Bool {
return bool
*/
}
throw DecodingError._typeMismatch(at: self.codingPath, expectation: type, reality: value)
}
fileprivate func unbox(_ value: Any, as type: Int.Type) throws -> Int? {
guard !(value is NSNull) else { return nil }
guard let number = value as? NSNumber else {
throw DecodingError._typeMismatch(at: self.codingPath, expectation: type, reality: value)
}
let int = number.intValue
guard NSNumber(value: int) == number else {
throw DecodingError.dataCorrupted(DecodingError.Context(codingPath: self.codingPath, debugDescription: "Parsed JSON number <\(number)> does not fit in \(type)."))
}
return int
}
fileprivate func unbox(_ value: Any, as type: Int8.Type) throws -> Int8? {
guard !(value is NSNull) else { return nil }
guard let number = value as? NSNumber else {
throw DecodingError._typeMismatch(at: self.codingPath, expectation: type, reality: value)
}
let int8 = number.int8Value
guard NSNumber(value: int8) == number else {
throw DecodingError.dataCorrupted(DecodingError.Context(codingPath: self.codingPath, debugDescription: "Parsed JSON number <\(number)> does not fit in \(type)."))
}
return int8
}
fileprivate func unbox(_ value: Any, as type: Int16.Type) throws -> Int16? {
guard !(value is NSNull) else { return nil }
guard let number = value as? NSNumber else {
throw DecodingError._typeMismatch(at: self.codingPath, expectation: type, reality: value)
}
let int16 = number.int16Value
guard NSNumber(value: int16) == number else {
throw DecodingError.dataCorrupted(DecodingError.Context(codingPath: self.codingPath, debugDescription: "Parsed JSON number <\(number)> does not fit in \(type)."))
}
return int16
}
fileprivate func unbox(_ value: Any, as type: Int32.Type) throws -> Int32? {
guard !(value is NSNull) else { return nil }
guard let number = value as? NSNumber else {
throw DecodingError._typeMismatch(at: self.codingPath, expectation: type, reality: value)
}
let int32 = number.int32Value
guard NSNumber(value: int32) == number else {
throw DecodingError.dataCorrupted(DecodingError.Context(codingPath: self.codingPath, debugDescription: "Parsed JSON number <\(number)> does not fit in \(type)."))
}
return int32
}
fileprivate func unbox(_ value: Any, as type: Int64.Type) throws -> Int64? {
guard !(value is NSNull) else { return nil }
guard let number = value as? NSNumber else {
throw DecodingError._typeMismatch(at: self.codingPath, expectation: type, reality: value)
}
let int64 = number.int64Value
guard NSNumber(value: int64) == number else {
throw DecodingError.dataCorrupted(DecodingError.Context(codingPath: self.codingPath, debugDescription: "Parsed JSON number <\(number)> does not fit in \(type)."))
}
return int64
}
fileprivate func unbox(_ value: Any, as type: UInt.Type) throws -> UInt? {
guard !(value is NSNull) else { return nil }
guard let number = value as? NSNumber else {
throw DecodingError._typeMismatch(at: self.codingPath, expectation: type, reality: value)
}
let uint = number.uintValue
guard NSNumber(value: uint) == number else {
throw DecodingError.dataCorrupted(DecodingError.Context(codingPath: self.codingPath, debugDescription: "Parsed JSON number <\(number)> does not fit in \(type)."))
}
return uint
}
fileprivate func unbox(_ value: Any, as type: UInt8.Type) throws -> UInt8? {
guard !(value is NSNull) else { return nil }
guard let number = value as? NSNumber else {
throw DecodingError._typeMismatch(at: self.codingPath, expectation: type, reality: value)
}
let uint8 = number.uint8Value
guard NSNumber(value: uint8) == number else {
throw DecodingError.dataCorrupted(DecodingError.Context(codingPath: self.codingPath, debugDescription: "Parsed JSON number <\(number)> does not fit in \(type)."))
}
return uint8
}
fileprivate func unbox(_ value: Any, as type: UInt16.Type) throws -> UInt16? {
guard !(value is NSNull) else { return nil }
guard let number = value as? NSNumber else {
throw DecodingError._typeMismatch(at: self.codingPath, expectation: type, reality: value)
}
let uint16 = number.uint16Value
guard NSNumber(value: uint16) == number else {
throw DecodingError.dataCorrupted(DecodingError.Context(codingPath: self.codingPath, debugDescription: "Parsed JSON number <\(number)> does not fit in \(type)."))
}
return uint16
}
fileprivate func unbox(_ value: Any, as type: UInt32.Type) throws -> UInt32? {
guard !(value is NSNull) else { return nil }
guard let number = value as? NSNumber else {
throw DecodingError._typeMismatch(at: self.codingPath, expectation: type, reality: value)
}
let uint32 = number.uint32Value
guard NSNumber(value: uint32) == number else {
throw DecodingError.dataCorrupted(DecodingError.Context(codingPath: self.codingPath, debugDescription: "Parsed JSON number <\(number)> does not fit in \(type)."))
}
return uint32
}
fileprivate func unbox(_ value: Any, as type: UInt64.Type) throws -> UInt64? {
guard !(value is NSNull) else { return nil }
guard let number = value as? NSNumber else {
throw DecodingError._typeMismatch(at: self.codingPath, expectation: type, reality: value)
}
let uint64 = number.uint64Value
guard NSNumber(value: uint64) == number else {
throw DecodingError.dataCorrupted(DecodingError.Context(codingPath: self.codingPath, debugDescription: "Parsed JSON number <\(number)> does not fit in \(type)."))
}
return uint64
}
fileprivate func unbox(_ value: Any, as type: Float.Type) throws -> Float? {
guard !(value is NSNull) else { return nil }
if let number = value as? NSNumber {
// We are willing to return a Float by losing precision:
// * If the original value was integral,
// * and the integral value was > Float.greatestFiniteMagnitude, we will fail
// * and the integral value was <= Float.greatestFiniteMagnitude, we are willing to lose precision past 2^24
// * If it was a Float, you will get back the precise value
// * If it was a Double or Decimal, you will get back the nearest approximation if it will fit
let double = number.doubleValue
guard abs(double) <= Double(Float.greatestFiniteMagnitude) else {
throw DecodingError.dataCorrupted(DecodingError.Context(codingPath: self.codingPath, debugDescription: "Parsed JSON number \(number) does not fit in \(type)."))
}
return Float(double)
/* FIXME: If swift-corelibs-foundation doesn't change to use NSNumber, this code path will need to be included and tested:
} else if let double = value as? Double {
if abs(double) <= Double(Float.max) {
return Float(double)
}
overflow = true
} else if let int = value as? Int {
if let float = Float(exactly: int) {
return float
}
overflow = true
*/
} else if let string = value as? String,
case .convertFromString(let posInfString, let negInfString, let nanString) = self.options.nonConformingFloatDecodingStrategy {
if string == posInfString {
return Float.infinity
} else if string == negInfString {
return -Float.infinity
} else if string == nanString {
return Float.nan
}
}
throw DecodingError._typeMismatch(at: self.codingPath, expectation: type, reality: value)
}
fileprivate func unbox(_ value: Any, as type: Double.Type) throws -> Double? {
guard !(value is NSNull) else { return nil }
if let number = value as? NSNumber {
// We are always willing to return the number as a Double:
// * If the original value was integral, it is guaranteed to fit in a Double; we are willing to lose precision past 2^53 if you encoded a UInt64 but requested a Double
// * If it was a Float or Double, you will get back the precise value
// * If it was Decimal, you will get back the nearest approximation
return number.doubleValue
/* FIXME: If swift-corelibs-foundation doesn't change to use NSNumber, this code path will need to be included and tested:
} else if let double = value as? Double {
return double
} else if let int = value as? Int {
if let double = Double(exactly: int) {
return double
}
overflow = true
*/
} else if let string = value as? String,
case .convertFromString(let posInfString, let negInfString, let nanString) = self.options.nonConformingFloatDecodingStrategy {
if string == posInfString {
return Double.infinity
} else if string == negInfString {
return -Double.infinity
} else if string == nanString {
return Double.nan
}
}
throw DecodingError._typeMismatch(at: self.codingPath, expectation: type, reality: value)
}
fileprivate func unbox(_ value: Any, as type: String.Type) throws -> String? {
guard !(value is NSNull) else { return nil }
guard let string = value as? String else {
throw DecodingError._typeMismatch(at: self.codingPath, expectation: type, reality: value)
}
return string
}
fileprivate func unbox(_ value: Any, as type: Date.Type) throws -> Date? {
guard !(value is NSNull) else { return nil }
switch self.options.dateDecodingStrategy {
case .deferredToDate:
self.storage.push(container: value)
let date = try Date(from: self)
self.storage.popContainer()
return date
case .secondsSince1970:
let double = try self.unbox(value, as: Double.self)!
return Date(timeIntervalSince1970: double)
case .millisecondsSince1970:
let double = try self.unbox(value, as: Double.self)!
return Date(timeIntervalSince1970: double / 1000.0)
case .iso8601:
if #available(OSX 10.12, iOS 10.0, watchOS 3.0, tvOS 10.0, *) {
let string = try self.unbox(value, as: String.self)!
guard let date = _iso8601Formatter.date(from: string) else {
throw DecodingError.dataCorrupted(DecodingError.Context(codingPath: self.codingPath, debugDescription: "Expected date string to be ISO8601-formatted."))
}
return date
} else {
fatalError("ISO8601DateFormatter is unavailable on this platform.")
}
case .formatted(let formatter):
let string = try self.unbox(value, as: String.self)!
guard let date = formatter.date(from: string) else {
throw DecodingError.dataCorrupted(DecodingError.Context(codingPath: self.codingPath, debugDescription: "Date string does not match format expected by formatter."))
}
return date
case .custom(let closure):
self.storage.push(container: value)
let date = try closure(self)
self.storage.popContainer()
return date
}
}
fileprivate func unbox(_ value: Any, as type: Data.Type) throws -> Data? {
guard !(value is NSNull) else { return nil }
switch self.options.dataDecodingStrategy {
case .deferredToData:
self.storage.push(container: value)
let data = try Data(from: self)
self.storage.popContainer()
return data
case .base64:
guard let string = value as? String else {
throw DecodingError._typeMismatch(at: self.codingPath, expectation: type, reality: value)
}
guard let data = Data(base64Encoded: string) else {
throw DecodingError.dataCorrupted(DecodingError.Context(codingPath: self.codingPath, debugDescription: "Encountered Data is not valid Base64."))
}
return data
case .custom(let closure):
self.storage.push(container: value)
let data = try closure(self)
self.storage.popContainer()
return data
}
}
fileprivate func unbox(_ value: Any, as type: Decimal.Type) throws -> Decimal? {
guard !(value is NSNull) else { return nil }
// Attempt to bridge from NSDecimalNumber.
if let decimal = value as? Decimal {
return decimal
} else {
let doubleValue = try self.unbox(value, as: Double.self)!
return Decimal(doubleValue)
}
}
fileprivate func unbox<T : Decodable>(_ value: Any, as type: T.Type) throws -> T? {
let decoded: T
if T.self == Date.self {
guard let date = try self.unbox(value, as: Date.self) else { return nil }
decoded = date as! T
} else if T.self == Data.self {
guard let data = try self.unbox(value, as: Data.self) else { return nil }
decoded = data as! T
} else if T.self == URL.self {
guard let urlString = try self.unbox(value, as: String.self) else {
return nil
}
guard let url = URL(string: urlString) else {
throw DecodingError.dataCorrupted(DecodingError.Context(codingPath: self.codingPath,
debugDescription: "Invalid URL string."))
}
decoded = (url as! T)
} else if T.self == Decimal.self {
guard let decimal = try self.unbox(value, as: Decimal.self) else { return nil }
decoded = decimal as! T
} else {
self.storage.push(container: value)
decoded = try T(from: self)
self.storage.popContainer()
}
return decoded
}
}
//===----------------------------------------------------------------------===//
// Shared Key Types
//===----------------------------------------------------------------------===//
fileprivate struct _JSONKey : CodingKey {
public var stringValue: String
public var intValue: Int?
public init?(stringValue: String) {
self.stringValue = stringValue
self.intValue = nil
}
public init?(intValue: Int) {
self.stringValue = "\(intValue)"
self.intValue = intValue
}
fileprivate init(index: Int) {
self.stringValue = "Index \(index)"
self.intValue = index
}
fileprivate static let `super` = _JSONKey(stringValue: "super")!
}
//===----------------------------------------------------------------------===//
// Shared ISO8601 Date Formatter
//===----------------------------------------------------------------------===//
// NOTE: This value is implicitly lazy and _must_ be lazy. We're compiled against the latest SDK (w/ ISO8601DateFormatter), but linked against whichever Foundation the user has. ISO8601DateFormatter might not exist, so we better not hit this code path on an older OS.
@available(OSX 10.12, iOS 10.0, watchOS 3.0, tvOS 10.0, *)
fileprivate var _iso8601Formatter: ISO8601DateFormatter = {
let formatter = ISO8601DateFormatter()
formatter.formatOptions = .withInternetDateTime
return formatter
}()
//===----------------------------------------------------------------------===//
// Error Utilities
//===----------------------------------------------------------------------===//
fileprivate extension EncodingError {
/// Returns a `.invalidValue` error describing the given invalid floating-point value.
///
///
/// - parameter value: The value that was invalid to encode.
/// - parameter path: The path of `CodingKey`s taken to encode this value.
/// - returns: An `EncodingError` with the appropriate path and debug description.
fileprivate static func _invalidFloatingPointValue<T : FloatingPoint>(_ value: T, at codingPath: [CodingKey]) -> EncodingError {
let valueDescription: String
if value == T.infinity {
valueDescription = "\(T.self).infinity"
} else if value == -T.infinity {
valueDescription = "-\(T.self).infinity"
} else {
valueDescription = "\(T.self).nan"
}
let debugDescription = "Unable to encode \(valueDescription) directly in JSON. Use JSONEncoder.NonConformingFloatEncodingStrategy.convertToString to specify how the value should be encoded."
return .invalidValue(value, EncodingError.Context(codingPath: codingPath, debugDescription: debugDescription))
}
}
| apache-2.0 | 299403c851e7ccec8906f1a329bc67d6 | 42.589556 | 267 | 0.650595 | 4.936501 | false | false | false | false |
NordicSemiconductor/IOS-Pods-DFU-Library | Example/Pods/ZIPFoundation/Sources/ZIPFoundation/Data+Serialization.swift | 1 | 3597 | //
// Data+Serialization.swift
// ZIPFoundation
//
// Copyright © 2017-2020 Thomas Zoechling, https://www.peakstep.com and the ZIP Foundation project authors.
// Released under the MIT License.
//
// See https://github.com/weichsel/ZIPFoundation/blob/master/LICENSE for license information.
//
import Foundation
protocol DataSerializable {
static var size: Int { get }
init?(data: Data, additionalDataProvider: (Int) throws -> Data)
var data: Data { get }
}
extension Data {
enum DataError: Error {
case unreadableFile
case unwritableFile
}
func scanValue<T>(start: Int) -> T {
let subdata = self.subdata(in: start..<start+MemoryLayout<T>.size)
#if swift(>=5.0)
return subdata.withUnsafeBytes { $0.load(as: T.self) }
#else
return subdata.withUnsafeBytes { $0.pointee }
#endif
}
static func readStruct<T>(from file: UnsafeMutablePointer<FILE>, at offset: Int) -> T? where T: DataSerializable {
fseek(file, offset, SEEK_SET)
guard let data = try? self.readChunk(of: T.size, from: file) else {
return nil
}
let structure = T(data: data, additionalDataProvider: { (additionalDataSize) -> Data in
return try self.readChunk(of: additionalDataSize, from: file)
})
return structure
}
static func consumePart(of size: Int, chunkSize: Int, skipCRC32: Bool = false,
provider: Provider, consumer: Consumer) throws -> CRC32 {
let readInOneChunk = (size < chunkSize)
var chunkSize = readInOneChunk ? size : chunkSize
var checksum = CRC32(0)
var bytesRead = 0
while bytesRead < size {
let remainingSize = size - bytesRead
chunkSize = remainingSize < chunkSize ? remainingSize : chunkSize
let data = try provider(bytesRead, chunkSize)
try consumer(data)
if !skipCRC32 {
checksum = data.crc32(checksum: checksum)
}
bytesRead += chunkSize
}
return checksum
}
static func readChunk(of size: Int, from file: UnsafeMutablePointer<FILE>) throws -> Data {
let alignment = MemoryLayout<UInt>.alignment
#if swift(>=4.1)
let bytes = UnsafeMutableRawPointer.allocate(byteCount: size, alignment: alignment)
#else
let bytes = UnsafeMutableRawPointer.allocate(bytes: size, alignedTo: alignment)
#endif
let bytesRead = fread(bytes, 1, size, file)
let error = ferror(file)
if error > 0 {
throw DataError.unreadableFile
}
#if swift(>=4.1)
return Data(bytesNoCopy: bytes, count: bytesRead, deallocator: .custom({ buf, _ in buf.deallocate() }))
#else
let deallocator = Deallocator.custom({ buf, _ in buf.deallocate(bytes: size, alignedTo: 1) })
return Data(bytesNoCopy: bytes, count: bytesRead, deallocator: deallocator)
#endif
}
static func write(chunk: Data, to file: UnsafeMutablePointer<FILE>) throws -> Int {
var sizeWritten = 0
chunk.withUnsafeBytes { (rawBufferPointer) in
if let baseAddress = rawBufferPointer.baseAddress, rawBufferPointer.count > 0 {
let pointer = baseAddress.assumingMemoryBound(to: UInt8.self)
sizeWritten = fwrite(pointer, 1, chunk.count, file)
}
}
let error = ferror(file)
if error > 0 {
throw DataError.unwritableFile
}
return sizeWritten
}
}
| bsd-3-clause | 03bed5267c95e76604a6ba9469ed5b12 | 35.693878 | 118 | 0.615128 | 4.423124 | false | false | false | false |
ioscreator/ioscreator | IOSCustomizeNavBarTutorial/IOSCustomizeNavBarTutorial/ViewController.swift | 1 | 950 | //
// ViewController.swift
// IOSCustomizeNavBarTutorial
//
// Created by Arthur Knopper on 16/02/2019.
// Copyright © 2019 Arthur Knopper. All rights reserved.
//
import UIKit
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
}
override func viewDidAppear(_ animated: Bool) {
// 1
let nav = self.navigationController?.navigationBar
// 2
nav?.barStyle = UIBarStyle.black
nav?.tintColor = UIColor.yellow
// 3
let imageView = UIImageView(frame: CGRect(x: 0, y: 0, width: 40, height: 40))
imageView.contentMode = .scaleAspectFit
// 4
let image = UIImage(named: "Apple_Swift_Logo")
imageView.image = image
// 5
navigationItem.titleView = imageView
}
}
| mit | ee042b0236b8cf00df6569385d67e042 | 22.725 | 85 | 0.599579 | 4.721393 | false | false | false | false |
zhugejunwei/LeetCode | 72. Edit Distance.swift | 1 | 820 | import Darwin
func minDistance(word1: String, _ word2: String) -> Int {
if word1.isEmpty { return word2.characters.count }
if word2.isEmpty { return word1.characters.count }
var aw1 = Array(word1.characters)
var aw2 = Array(word2.characters)
let l1 = aw1.count
let l2 = aw2.count
var count = Array(count: l1+1, repeatedValue: Array(count: l2+1, repeatedValue: 0))
for i in 0...l1 { count[i][0] = i }
for j in 1...l2 { count[0][j] = j }
for i in 1...l1 {
for j in 1...l2 {
if aw1[i-1] == aw2[j-1] {
count[i][j] = count[i-1][j-1]
}else {
count[i][j] = min(count[i-1][j-1], count[i][j-1], count[i-1][j]) + 1
}
}
}
return count[l1][l2]
}
var a = "rabpt"
var b = "habbot"
minDistance(a,b) | mit | 2732c3763dd1697cdc865b669ce8df6c | 26.366667 | 87 | 0.534146 | 2.760943 | false | false | false | false |
abertelrud/swift-package-manager | Tests/SourceControlTests/RepositoryManagerTests.swift | 2 | 35698 | //===----------------------------------------------------------------------===//
//
// This source file is part of the Swift open source project
//
// Copyright (c) 2014-2017 Apple Inc. and the Swift project authors
// Licensed under Apache License v2.0 with Runtime Library Exception
//
// See http://swift.org/LICENSE.txt for license information
// See http://swift.org/CONTRIBUTORS.txt for the list of Swift project authors
//
//===----------------------------------------------------------------------===//
@testable import Basics
import PackageModel
import SPMTestSupport
@testable import SourceControl
import TSCBasic
import XCTest
class RepositoryManagerTests: XCTestCase {
func testBasics() throws {
let fs = localFileSystem
let observability = ObservabilitySystem.makeForTesting()
try testWithTemporaryDirectory { path in
let provider = DummyRepositoryProvider(fileSystem: fs)
let delegate = DummyRepositoryManagerDelegate()
let manager = RepositoryManager(
fileSystem: fs,
path: path,
provider: provider,
delegate: delegate
)
let dummyRepo = RepositorySpecifier(path: .init(path: "/dummy"))
let badDummyRepo = RepositorySpecifier(path: .init(path: "/badDummy"))
var prevHandle: RepositoryManager.RepositoryHandle?
// Check that we can "fetch" a repository.
do {
delegate.prepare(fetchExpected: true, updateExpected: false)
let handle = try manager.lookup(repository: dummyRepo, observabilityScope: observability.topScope)
XCTAssertNoDiagnostics(observability.diagnostics)
prevHandle = handle
XCTAssertEqual(provider.numFetches, 0)
// Open the repository.
let repository = try! handle.open()
XCTAssertEqual(try! repository.getTags(), ["1.0.0"])
// Create a checkout of the repository.
let checkoutPath = path.appending(component: "checkout")
_ = try! handle.createWorkingCopy(at: checkoutPath, editable: false)
XCTAssertDirectoryExists(checkoutPath)
XCTAssertFileExists(checkoutPath.appending(component: "README.txt"))
try delegate.wait(timeout: .now() + 2)
XCTAssertEqual(delegate.willFetch.map { $0.repository }, [dummyRepo])
XCTAssertEqual(delegate.didFetch.map { $0.repository }, [dummyRepo])
}
// Get a bad repository.
do {
delegate.prepare(fetchExpected: true, updateExpected: false)
XCTAssertThrowsError(try manager.lookup(repository: badDummyRepo, observabilityScope: observability.topScope)) { error in
XCTAssertEqual(error as? DummyError, DummyError.invalidRepository)
}
XCTAssertNotNil(prevHandle)
try delegate.wait(timeout: .now() + 2)
XCTAssertEqual(delegate.willFetch.map { $0.repository }, [dummyRepo, badDummyRepo])
XCTAssertEqual(delegate.didFetch.map { $0.repository }, [dummyRepo, badDummyRepo])
// We shouldn't have made any update call yet.
XCTAssert(delegate.willUpdate.isEmpty)
XCTAssert(delegate.didUpdate.isEmpty)
}
do {
delegate.prepare(fetchExpected: false, updateExpected: true)
let handle = try manager.lookup(repository: dummyRepo, observabilityScope: observability.topScope)
XCTAssertNoDiagnostics(observability.diagnostics)
XCTAssertEqual(handle.repository, dummyRepo)
XCTAssertEqual(handle.repository, prevHandle?.repository)
// We should always get back the same handle once fetched.
// Since we looked up this repo again, we should have made a fetch call.
try delegate.wait(timeout: .now() + 2)
XCTAssertEqual(provider.numFetches, 1)
XCTAssertEqual(delegate.willUpdate, [dummyRepo])
XCTAssertEqual(delegate.didUpdate, [dummyRepo])
}
// Remove the repo.
do {
try manager.remove(repository: dummyRepo)
// Check removing the repo updates the persistent file.
/*do {
let checkoutsStateFile = path.appending(component: "checkouts-state.json")
let jsonData = try JSON(bytes: localFileSystem.readFileContents(checkoutsStateFile))
XCTAssertEqual(jsonData.dictionary?["object"]?.dictionary?["repositories"]?.dictionary?[dummyRepo.location.description], nil)
}*/
// We should get a new handle now because we deleted the existing repository.
delegate.prepare(fetchExpected: true, updateExpected: false)
let handle = try manager.lookup(repository: dummyRepo, observabilityScope: observability.topScope)
XCTAssertNoDiagnostics(observability.diagnostics)
XCTAssertEqual(handle.repository, dummyRepo)
// We should have tried fetching these two.
try delegate.wait(timeout: .now() + 2)
XCTAssertEqual(delegate.willFetch.map { $0.repository }, [dummyRepo, badDummyRepo, dummyRepo])
XCTAssertEqual(delegate.didFetch.map { $0.repository }, [dummyRepo, badDummyRepo, dummyRepo])
XCTAssertEqual(delegate.willUpdate, [dummyRepo])
XCTAssertEqual(delegate.didUpdate, [dummyRepo])
}
}
}
func testCache() throws {
if #available(macOS 11, *) {
// No need to skip the test.
}
else {
// Avoid a crasher that seems to happen only on macOS 10.15, but leave an environment variable for testing.
try XCTSkipUnless(ProcessEnv.vars["SWIFTPM_ENABLE_FLAKY_REPOSITORYMANAGERTESTS"] == "1", "skipping test that sometimes crashes in CI (rdar://70540298)")
}
let fs = localFileSystem
let observability = ObservabilitySystem.makeForTesting()
try fixture(name: "DependencyResolution/External/Simple") { fixturePath in
let cachePath = fixturePath.appending(component: "cache")
let repositoriesPath = fixturePath.appending(component: "repositories")
let repo = RepositorySpecifier(path: fixturePath.appending(component: "Foo"))
let provider = GitRepositoryProvider()
let delegate = DummyRepositoryManagerDelegate()
let manager = RepositoryManager(
fileSystem: fs,
path: repositoriesPath,
provider: provider,
cachePath: cachePath,
cacheLocalPackages: true,
delegate: delegate
)
// fetch packages and populate cache
delegate.prepare(fetchExpected: true, updateExpected: false)
_ = try manager.lookup(repository: repo, observabilityScope: observability.topScope)
XCTAssertNoDiagnostics(observability.diagnostics)
XCTAssertDirectoryExists(cachePath.appending(repo.storagePath()))
XCTAssertDirectoryExists(repositoriesPath.appending(repo.storagePath()))
try delegate.wait(timeout: .now() + 2)
XCTAssertEqual(delegate.willFetch[0].details,
RepositoryManager.FetchDetails(fromCache: false, updatedCache: false))
XCTAssertEqual(try delegate.didFetch[0].result.get(),
RepositoryManager.FetchDetails(fromCache: false, updatedCache: true))
// removing the repositories path to force re-fetch
try fs.removeFileTree(repositoriesPath)
// fetch packages from the cache
delegate.prepare(fetchExpected: true, updateExpected: false)
_ = try manager.lookup(repository: repo, observabilityScope: observability.topScope)
XCTAssertNoDiagnostics(observability.diagnostics)
XCTAssertDirectoryExists(repositoriesPath.appending(repo.storagePath()))
try delegate.wait(timeout: .now() + 2)
XCTAssertEqual(delegate.willFetch[1].details,
RepositoryManager.FetchDetails(fromCache: true, updatedCache: false))
XCTAssertEqual(try delegate.didFetch[1].result.get(),
RepositoryManager.FetchDetails(fromCache: true, updatedCache: true))
// reset the state on disk
try fs.removeFileTree(cachePath)
try fs.removeFileTree(repositoriesPath)
// fetch packages and populate cache
delegate.prepare(fetchExpected: true, updateExpected: false)
_ = try manager.lookup(repository: repo, observabilityScope: observability.topScope)
XCTAssertNoDiagnostics(observability.diagnostics)
XCTAssertDirectoryExists(cachePath.appending(repo.storagePath()))
XCTAssertDirectoryExists(repositoriesPath.appending(repo.storagePath()))
try delegate.wait(timeout: .now() + 2)
XCTAssertEqual(delegate.willFetch[2].details,
RepositoryManager.FetchDetails(fromCache: false, updatedCache: false))
XCTAssertEqual(try delegate.didFetch[2].result.get(),
RepositoryManager.FetchDetails(fromCache: false, updatedCache: true))
// update packages from the cache
delegate.prepare(fetchExpected: false, updateExpected: true)
_ = try manager.lookup(repository: repo, observabilityScope: observability.topScope)
XCTAssertNoDiagnostics(observability.diagnostics)
try delegate.wait(timeout: .now() + 2)
XCTAssertEqual(delegate.willUpdate[0].storagePath(), repo.storagePath())
XCTAssertEqual(delegate.didUpdate[0].storagePath(), repo.storagePath())
}
}
func testReset() throws {
let fs = localFileSystem
let observability = ObservabilitySystem.makeForTesting()
try testWithTemporaryDirectory { path in
let repos = path.appending(component: "repo")
let provider = DummyRepositoryProvider(fileSystem: fs)
let delegate = DummyRepositoryManagerDelegate()
try fs.createDirectory(repos, recursive: true)
let manager = RepositoryManager(
fileSystem: fs,
path: repos,
provider: provider,
delegate: delegate
)
let dummyRepo = RepositorySpecifier(path: .init(path: "/dummy"))
delegate.prepare(fetchExpected: true, updateExpected: false)
_ = try manager.lookup(repository: dummyRepo, observabilityScope: observability.topScope)
XCTAssertNoDiagnostics(observability.diagnostics)
delegate.prepare(fetchExpected: false, updateExpected: true)
_ = try manager.lookup(repository: dummyRepo, observabilityScope: observability.topScope)
XCTAssertNoDiagnostics(observability.diagnostics)
try delegate.wait(timeout: .now() + 2)
XCTAssertEqual(delegate.willFetch.count, 1)
XCTAssertEqual(delegate.didFetch.count, 1)
try manager.reset()
XCTAssertTrue(!fs.isDirectory(repos))
try fs.createDirectory(repos, recursive: true)
delegate.prepare(fetchExpected: true, updateExpected: false)
_ = try manager.lookup(repository: dummyRepo, observabilityScope: observability.topScope)
XCTAssertNoDiagnostics(observability.diagnostics)
try delegate.wait(timeout: .now() + 2)
XCTAssertEqual(delegate.willFetch.count, 2)
XCTAssertEqual(delegate.didFetch.count, 2)
}
}
/// Check that the manager is persistent.
func testPersistence() throws {
let fs = localFileSystem
let observability = ObservabilitySystem.makeForTesting()
try testWithTemporaryDirectory { path in
let provider = DummyRepositoryProvider(fileSystem: fs)
let dummyRepo = RepositorySpecifier(path: .init(path: "/dummy"))
// Do the initial fetch.
do {
let delegate = DummyRepositoryManagerDelegate()
let manager = RepositoryManager(
fileSystem: fs,
path: path,
provider: provider,
delegate: delegate
)
delegate.prepare(fetchExpected: true, updateExpected: false)
_ = try manager.lookup(repository: dummyRepo, observabilityScope: observability.topScope)
XCTAssertNoDiagnostics(observability.diagnostics)
try delegate.wait(timeout: .now() + 2)
XCTAssertEqual(delegate.willFetch.map { $0.repository }, [dummyRepo])
XCTAssertEqual(delegate.didFetch.map { $0.repository }, [dummyRepo])
}
// We should have performed one fetch.
XCTAssertEqual(provider.numClones, 1)
XCTAssertEqual(provider.numFetches, 0)
// Create a new manager, and fetch.
do {
let delegate = DummyRepositoryManagerDelegate()
let manager = RepositoryManager(
fileSystem: fs,
path: path,
provider: provider,
delegate: delegate
)
delegate.prepare(fetchExpected: true, updateExpected: false)
_ = try manager.lookup(repository: dummyRepo, observabilityScope: observability.topScope)
XCTAssertNoDiagnostics(observability.diagnostics)
// This time fetch shouldn't be called.
try delegate.wait(timeout: .now() + 2)
XCTAssertEqual(delegate.willFetch.map { $0.repository }, [])
}
// We shouldn't have done a new fetch.
XCTAssertEqual(provider.numClones, 1)
XCTAssertEqual(provider.numFetches, 1)
// Manually destroy the manager state, and check it still works.
do {
let delegate = DummyRepositoryManagerDelegate()
var manager = RepositoryManager(
fileSystem: fs,
path: path,
provider: provider,
delegate: delegate
)
try! fs.removeFileTree(path.appending(dummyRepo.storagePath()))
manager = RepositoryManager(
fileSystem: fs,
path: path,
provider: provider,
delegate: delegate
)
let dummyRepo = RepositorySpecifier(path: .init(path: "/dummy"))
delegate.prepare(fetchExpected: true, updateExpected: false)
_ = try manager.lookup(repository: dummyRepo, observabilityScope: observability.topScope)
XCTAssertNoDiagnostics(observability.diagnostics)
try delegate.wait(timeout: .now() + 2)
XCTAssertEqual(delegate.willFetch.map { $0.repository }, [dummyRepo])
XCTAssertEqual(delegate.didFetch.map { $0.repository }, [dummyRepo])
}
// We should have re-fetched.
XCTAssertEqual(provider.numClones, 2)
XCTAssertEqual(provider.numFetches, 1)
}
}
func testConcurrency() throws {
let fs = localFileSystem
let observability = ObservabilitySystem.makeForTesting()
try testWithTemporaryDirectory { path in
let provider = DummyRepositoryProvider(fileSystem: fs)
let delegate = DummyRepositoryManagerDelegate()
let manager = RepositoryManager(
fileSystem: fs,
path: path,
provider: provider,
delegate: delegate
)
let dummyRepo = RepositorySpecifier(path: .init(path: "/dummy"))
let group = DispatchGroup()
let results = ThreadSafeKeyValueStore<Int, Result<RepositoryManager.RepositoryHandle, Error>>()
let concurrency = 10000
for index in 0 ..< concurrency {
group.enter()
delegate.prepare(fetchExpected: index == 0, updateExpected: index > 0)
manager.lookup(
package: .init(url: dummyRepo.url),
repository: dummyRepo,
skipUpdate: false,
observabilityScope: observability.topScope,
delegateQueue: .sharedConcurrent,
callbackQueue: .sharedConcurrent
) { result in
results[index] = result
group.leave()
}
}
if case .timedOut = group.wait(timeout: .now() + 60) {
return XCTFail("timeout")
}
XCTAssertNoDiagnostics(observability.diagnostics)
try delegate.wait(timeout: .now() + 2)
XCTAssertEqual(delegate.willFetch.count, 1)
XCTAssertEqual(delegate.didFetch.count, 1)
XCTAssertEqual(delegate.willUpdate.count, concurrency - 1)
XCTAssertEqual(delegate.didUpdate.count, concurrency - 1)
XCTAssertEqual(results.count, concurrency)
for index in 0 ..< concurrency {
XCTAssertEqual(try results[index]?.get().repository, dummyRepo)
}
}
}
func testSkipUpdate() throws {
let fs = localFileSystem
let observability = ObservabilitySystem.makeForTesting()
try testWithTemporaryDirectory { path in
let repos = path.appending(component: "repo")
let provider = DummyRepositoryProvider(fileSystem: fs)
let delegate = DummyRepositoryManagerDelegate()
try fs.createDirectory(repos, recursive: true)
let manager = RepositoryManager(
fileSystem: fs,
path: repos,
provider: provider,
delegate: delegate
)
let dummyRepo = RepositorySpecifier(path: .init(path: "/dummy"))
delegate.prepare(fetchExpected: true, updateExpected: false)
_ = try manager.lookup(repository: dummyRepo, observabilityScope: observability.topScope)
XCTAssertNoDiagnostics(observability.diagnostics)
try delegate.wait(timeout: .now() + 2)
XCTAssertEqual(delegate.willFetch.count, 1)
XCTAssertEqual(delegate.didFetch.count, 1)
XCTAssertEqual(delegate.willUpdate.count, 0)
XCTAssertEqual(delegate.didUpdate.count, 0)
delegate.prepare(fetchExpected: false, updateExpected: true)
_ = try manager.lookup(repository: dummyRepo, observabilityScope: observability.topScope)
XCTAssertNoDiagnostics(observability.diagnostics)
delegate.prepare(fetchExpected: false, updateExpected: true)
_ = try manager.lookup(repository: dummyRepo, observabilityScope: observability.topScope)
XCTAssertNoDiagnostics(observability.diagnostics)
try delegate.wait(timeout: .now() + 2)
XCTAssertEqual(delegate.willFetch.count, 1)
XCTAssertEqual(delegate.didFetch.count, 1)
XCTAssertEqual(delegate.willUpdate.count, 2)
XCTAssertEqual(delegate.didUpdate.count, 2)
delegate.prepare(fetchExpected: false, updateExpected: false)
_ = try manager.lookup(repository: dummyRepo, skipUpdate: true, observabilityScope: observability.topScope)
XCTAssertNoDiagnostics(observability.diagnostics)
try delegate.wait(timeout: .now() + 2)
XCTAssertEqual(delegate.willFetch.count, 1)
XCTAssertEqual(delegate.didFetch.count, 1)
XCTAssertEqual(delegate.willUpdate.count, 2)
XCTAssertEqual(delegate.didUpdate.count, 2)
}
}
func testCancel() throws {
let observability = ObservabilitySystem.makeForTesting()
let cancellator = Cancellator(observabilityScope: observability.topScope)
let total = 10
let provider = MockRepositoryProvider(total: total)
let manager = RepositoryManager(
fileSystem: InMemoryFileSystem(),
path: .root,
provider: provider,
maxConcurrentOperations: total
)
cancellator.register(name: "repository manager", handler: manager)
//let startGroup = DispatchGroup()
let finishGroup = DispatchGroup()
let results = ThreadSafeKeyValueStore<RepositorySpecifier, Result<RepositoryManager.RepositoryHandle, Error>>()
for index in 0 ..< total {
let repository = RepositorySpecifier(path: try .init(validating: "/repo/\(index)"))
provider.startGroup.enter()
finishGroup.enter()
manager.lookup(
package: .init(url: repository.url),
repository: repository,
skipUpdate: true,
observabilityScope: observability.topScope,
delegateQueue: .sharedConcurrent,
callbackQueue: .sharedConcurrent
) { result in
defer { finishGroup.leave() }
results[repository] = result
}
}
XCTAssertEqual(.success, provider.startGroup.wait(timeout: .now() + 5), "timeout starting tasks")
let cancelled = cancellator._cancel(deadline: .now() + .seconds(1))
XCTAssertEqual(cancelled, 1, "expected to be terminated")
XCTAssertNoDiagnostics(observability.diagnostics)
// this releases the fetch threads that are waiting to test if the call was cancelled
provider.terminatedGroup.leave()
XCTAssertEqual(.success, finishGroup.wait(timeout: .now() + 5), "timeout finishing tasks")
XCTAssertEqual(results.count, total, "expected \(total) results")
for (repository, result) in results.get() {
switch (Int(repository.basename)! < total / 2, result) {
case (true, .success):
break // as expected!
case (true, .failure(let error)):
XCTFail("expected success, but failed with \(type(of: error)) '\(error)'")
case (false, .success):
XCTFail("expected operation to be cancelled")
case (false, .failure(let error)):
XCTAssert(error is CancellationError, "expected error to be CancellationError, but was \(type(of: error)) '\(error)'")
}
}
// wait for outstanding threads that would be cancelled and completion handlers thrown away
XCTAssertEqual(.success, provider.outstandingGroup.wait(timeout: .now() + .seconds(5)), "timeout waiting for outstanding tasks")
// the provider called in a thread managed by the RepositoryManager
// the use of blocking semaphore is intentional
class MockRepositoryProvider: RepositoryProvider {
let total: Int
// this DispatchGroup is used to wait for the requests to start before calling cancel
let startGroup = DispatchGroup()
// this DispatchGroup is used to park the delayed threads that would be cancelled
let terminatedGroup = DispatchGroup()
// this DispatchGroup is used to monitor the outstanding threads that would be cancelled and completion handlers thrown away
let outstandingGroup = DispatchGroup()
init(total: Int) {
self.total = total
self.terminatedGroup.enter()
}
func fetch(repository: RepositorySpecifier, to path: AbsolutePath, progressHandler: ((FetchProgress) -> Void)?) throws {
print("fetching \(repository)")
// startGroup may not be 100% accurate given the blocking nature of the provider so giving it a bit of a buffer
DispatchQueue.sharedConcurrent.asyncAfter(deadline: .now() + .milliseconds(100)) {
self.startGroup.leave()
}
if Int(repository.basename)! >= total / 2 {
self.outstandingGroup.enter()
defer { self.outstandingGroup.leave() }
print("\(repository) waiting to be cancelled")
XCTAssertEqual(.success, self.terminatedGroup.wait(timeout: .now() + 5), "timeout waiting on terminated signal")
throw StringError("\(repository) should be cancelled")
}
print("\(repository) okay")
}
func repositoryExists(at path: AbsolutePath) throws -> Bool {
return false
}
func open(repository: RepositorySpecifier, at path: AbsolutePath) throws -> Repository {
fatalError("should not be called")
}
func createWorkingCopy(repository: RepositorySpecifier, sourcePath: AbsolutePath, at destinationPath: AbsolutePath, editable: Bool) throws -> WorkingCheckout {
fatalError("should not be called")
}
func workingCopyExists(at path: AbsolutePath) throws -> Bool {
fatalError("should not be called")
}
func openWorkingCopy(at path: AbsolutePath) throws -> WorkingCheckout {
fatalError("should not be called")
}
func copy(from sourcePath: AbsolutePath, to destinationPath: AbsolutePath) throws {
fatalError("should not be called")
}
func isValidDirectory(_ directory: AbsolutePath) -> Bool {
fatalError("should not be called")
}
func isValidRefFormat(_ ref: String) -> Bool {
fatalError("should not be called")
}
func cancel(deadline: DispatchTime) throws {
print("cancel")
}
}
}
}
extension RepositoryManager {
public convenience init(
fileSystem: FileSystem,
path: AbsolutePath,
provider: RepositoryProvider,
cachePath: AbsolutePath? = .none,
cacheLocalPackages: Bool = false,
maxConcurrentOperations: Int? = .none,
delegate: RepositoryManagerDelegate? = .none
) {
self.init(
fileSystem: fileSystem,
path: path,
provider: provider,
cachePath: cachePath,
cacheLocalPackages: cacheLocalPackages,
maxConcurrentOperations: maxConcurrentOperations,
initializationWarningHandler: { _ in },
delegate: delegate
)
}
fileprivate func lookup(repository: RepositorySpecifier, skipUpdate: Bool = false, observabilityScope: ObservabilityScope) throws -> RepositoryHandle {
return try tsc_await {
self.lookup(
package: .init(url: repository.url),
repository: repository,
skipUpdate: skipUpdate,
observabilityScope: observabilityScope,
delegateQueue: .sharedConcurrent,
callbackQueue: .sharedConcurrent,
completion: $0
)
}
}
}
private enum DummyError: Swift.Error {
case invalidRepository
}
private class DummyRepository: Repository {
unowned let provider: DummyRepositoryProvider
init(provider: DummyRepositoryProvider) {
self.provider = provider
}
func getTags() throws -> [String] {
["1.0.0"]
}
func resolveRevision(tag: String) throws -> Revision {
fatalError("unexpected API call")
}
func resolveRevision(identifier: String) throws -> Revision {
fatalError("unexpected API call")
}
func exists(revision: Revision) -> Bool {
fatalError("unexpected API call")
}
func isValidDirectory(_ directory: AbsolutePath) -> Bool {
fatalError("unexpected API call")
}
func isValidRefFormat(_ ref: String) -> Bool {
fatalError("unexpected API call")
}
func fetch() throws {
self.provider.increaseFetchCount()
}
func openFileView(revision: Revision) throws -> FileSystem {
fatalError("unexpected API call")
}
public func openFileView(tag: String) throws -> FileSystem {
fatalError("unexpected API call")
}
}
private class DummyRepositoryProvider: RepositoryProvider {
private let fileSystem: FileSystem
private let lock = NSLock()
private var _numClones = 0
private var _numFetches = 0
init(fileSystem: FileSystem) {
self.fileSystem = fileSystem
}
func fetch(repository: RepositorySpecifier, to path: AbsolutePath, progressHandler: FetchProgress.Handler? = nil) throws {
assert(!self.fileSystem.exists(path))
try self.fileSystem.createDirectory(path, recursive: true)
try self.fileSystem.writeFileContents(path.appending(component: "readme.md"), bytes: ByteString(encodingAsUTF8: repository.location.description))
self.lock.withLock {
self._numClones += 1
}
// We only support one dummy URL.
let basename = repository.url.pathComponents.last!
if basename != "dummy" {
throw DummyError.invalidRepository
}
}
public func repositoryExists(at path: AbsolutePath) throws -> Bool {
return self.fileSystem.isDirectory(path)
}
func copy(from sourcePath: AbsolutePath, to destinationPath: AbsolutePath) throws {
try self.fileSystem.copy(from: sourcePath, to: destinationPath)
self.lock.withLock {
self._numClones += 1
}
// We only support one dummy URL.
let basename = sourcePath.basename
if basename != "dummy" {
throw DummyError.invalidRepository
}
}
func open(repository: RepositorySpecifier, at path: AbsolutePath) -> Repository {
return DummyRepository(provider: self)
}
func createWorkingCopy(repository: RepositorySpecifier, sourcePath: AbsolutePath, at destinationPath: AbsolutePath, editable: Bool) throws -> WorkingCheckout {
try self.fileSystem.createDirectory(destinationPath)
try self.fileSystem.writeFileContents(destinationPath.appending(component: "README.txt"), bytes: "Hi")
return try self.openWorkingCopy(at: destinationPath)
}
func workingCopyExists(at path: AbsolutePath) throws -> Bool {
return false
}
func openWorkingCopy(at path: AbsolutePath) throws -> WorkingCheckout {
return DummyWorkingCheckout(at: path)
}
func isValidDirectory(_ directory: AbsolutePath) -> Bool {
return true
}
func isValidRefFormat(_ ref: String) -> Bool {
return true
}
func cancel(deadline: DispatchTime) throws {
// noop
}
func increaseFetchCount() {
self.lock.withLock {
self._numFetches += 1
}
}
var numClones: Int {
self.lock.withLock {
self._numClones
}
}
var numFetches: Int {
self.lock.withLock {
self._numFetches
}
}
struct DummyWorkingCheckout: WorkingCheckout {
let path : AbsolutePath
init(at path: AbsolutePath) {
self.path = path
}
func getTags() throws -> [String] {
fatalError("not implemented")
}
func getCurrentRevision() throws -> Revision {
fatalError("not implemented")
}
func fetch() throws {
fatalError("not implemented")
}
func hasUnpushedCommits() throws -> Bool {
fatalError("not implemented")
}
func hasUncommittedChanges() -> Bool {
fatalError("not implemented")
}
func checkout(tag: String) throws {
fatalError("not implemented")
}
func checkout(revision: Revision) throws {
fatalError("not implemented")
}
func exists(revision: Revision) -> Bool {
fatalError("not implemented")
}
func checkout(newBranch: String) throws {
fatalError("not implemented")
}
func isAlternateObjectStoreValid() -> Bool {
fatalError("not implemented")
}
func areIgnored(_ paths: [AbsolutePath]) throws -> [Bool] {
fatalError("not implemented")
}
}
}
private class DummyRepositoryManagerDelegate: RepositoryManager.Delegate {
private var _willFetch = ThreadSafeArrayStore<(repository: RepositorySpecifier, details: RepositoryManager.FetchDetails)>()
private var _didFetch = ThreadSafeArrayStore<(repository: RepositorySpecifier, result: Result<RepositoryManager.FetchDetails, Error>)>()
private var _willUpdate = ThreadSafeArrayStore<RepositorySpecifier>()
private var _didUpdate = ThreadSafeArrayStore<RepositorySpecifier>()
private var group = DispatchGroup()
public func prepare(fetchExpected: Bool, updateExpected: Bool) {
if fetchExpected {
self.group.enter() // will fetch
self.group.enter() // did fetch
}
if updateExpected {
self.group.enter() // will update
self.group.enter() // did v
}
}
public func reset() {
self.group = DispatchGroup()
self._willFetch = .init()
self._didFetch = .init()
self._willUpdate = .init()
self._didUpdate = .init()
}
public func wait(timeout: DispatchTime) throws {
switch self.group.wait(timeout: timeout) {
case .success:
return
case .timedOut:
throw StringError("timeout")
}
}
var willFetch: [(repository: RepositorySpecifier, details: RepositoryManager.FetchDetails)] {
return self._willFetch.get()
}
var didFetch: [(repository: RepositorySpecifier, result: Result<RepositoryManager.FetchDetails, Error>)] {
return self._didFetch.get()
}
var willUpdate: [RepositorySpecifier] {
return self._willUpdate.get()
}
var didUpdate: [RepositorySpecifier] {
return self._didUpdate.get()
}
func willFetch(package: PackageIdentity, repository: RepositorySpecifier, details: RepositoryManager.FetchDetails) {
self._willFetch.append((repository: repository, details: details))
self.group.leave()
}
func fetching(package: PackageIdentity, repository: RepositorySpecifier, objectsFetched: Int, totalObjectsToFetch: Int) {
}
func didFetch(package: PackageIdentity, repository: RepositorySpecifier, result: Result<RepositoryManager.FetchDetails, Error>, duration: DispatchTimeInterval) {
self._didFetch.append((repository: repository, result: result))
self.group.leave()
}
func willUpdate(package: PackageIdentity, repository: RepositorySpecifier) {
self._willUpdate.append(repository)
self.group.leave()
}
func didUpdate(package: PackageIdentity, repository: RepositorySpecifier, duration: DispatchTimeInterval) {
self._didUpdate.append(repository)
self.group.leave()
}
}
| apache-2.0 | 02337daba57bd735618904d87d3af3b6 | 39.938073 | 171 | 0.610819 | 5.682585 | false | false | false | false |
Shivam0911/IOS-Training-Projects | ListViewToGridViewConverter/ListViewToGridViewConverter/listViewCell.swift | 2 | 848 | //
// listViewCell.swift
// ListViewToGridViewConverter
//
// Created by MAC on 13/02/17.
// Copyright © 2017 Appinventiv. All rights reserved.
//
import UIKit
class listViewCell: UICollectionViewCell {
@IBOutlet weak var carImage: UIImageView!
@IBOutlet weak var carName: UILabel!
override func awakeFromNib() {
super.awakeFromNib()
// carImage.layer.cornerRadius = carImage.layer.bounds.height/2
// Initialization code
}
func populateTheData(_ data : [String:String]){
print(#function)
let imageUrl = data["CarImage"]!
self.carImage.backgroundColor = UIColor(patternImage: UIImage(named: imageUrl)!)
// carImage.backgroundColor = UIColor.cyan
carName.text = data["CarName"]!
}
override func prepareForReuse() {
}
}
| mit | dc56d060130081702e88cdb436bd9b78 | 24.666667 | 88 | 0.644628 | 4.505319 | false | false | false | false |
Pluto-Y/SwiftyEcharts | SwiftyEcharts/Models/Type/ItemStyle.swift | 1 | 4155 | //
// ItemStyle.swift
// SwiftyEcharts
//
// Created by Pluto Y on 03/01/2017.
// Copyright © 2017 com.pluto-y. All rights reserved.
//
public protocol ItemStyleContent: Colorful, Borderable, Shadowable, Opacitable {
var borderType: LineType? { get set }
}
public final class CommonItemStyleContent: ItemStyleContent {
public var color: Color?
public var color0: Color? // 目前只针对 CandlestickSerie, 阴线 图形的颜色。
public var borderColor: Color?
public var borderColor0: Color? // 目前只针对 CandlestickSerie, 阴线 图形的描边颜色。
public var borderWidth: Float?
public var borderType: LineType?
public var shadowBlur: Float?
public var shadowColor: Color?
public var shadowOffsetX: Float?
public var shadowOffsetY: Float?
public var opacity: Float? {
didSet {
validateOpacity()
}
}
/// 目前只针对 `BarSerie`
public var barBorderRadius: Float?
public var areaColor: Color?
public init() { }
}
extension CommonItemStyleContent: Enumable {
public enum Enums {
case color(Color), color0(Color), borderColor(Color), borderColor0(Color), borderWidth(Float), borderType(LineType), shadowBlur(Float), shadowColor(Color), shadowOffsetX(Float), shadowOffsetY(Float), opacity(Float), barBorderRadius(Float), areaColor(Color)
}
public typealias ContentEnum = Enums
public convenience init(_ elements: Enums...) {
self.init()
for ele in elements {
switch ele {
case let .color(color):
self.color = color
case let .color0(color0):
self.color0 = color0
case let .borderColor(color):
self.borderColor = color
case let .borderColor0(color0):
self.borderColor0 = color0
case let .borderWidth(width):
self.borderWidth = width
case let .borderType(borderType):
self.borderType = borderType
case let .shadowBlur(blur):
self.shadowBlur = blur
case let .shadowColor(color):
self.shadowColor = color
case let .shadowOffsetX(x):
self.shadowOffsetX = x
case let .shadowOffsetY(y):
self.shadowOffsetY = y
case let .opacity(opacity):
self.opacity = opacity
case let .barBorderRadius(barBorderRadius):
self.barBorderRadius = barBorderRadius
case let .areaColor(areaColor):
self.areaColor = areaColor
}
}
}
}
extension CommonItemStyleContent: Mappable {
public func mapping(_ map: Mapper) {
map["color"] = color
map["color0"] = color0
map["borderColor"] = borderColor
map["borderColor0"] = borderColor0
map["borderWidth"] = borderWidth
map["borderType"] = borderType
map["shadowBlur"] = shadowBlur
map["shadowColor"] = shadowColor
map["shadowOffsetX"] = shadowOffsetX
map["shadowOffsetY"] = shadowOffsetY
map["opacity"] = opacity
map["barBorderRadius"] = barBorderRadius
map["areaColor"] = areaColor
}
}
public final class ItemStyle: Emphasisable {
public typealias Style = CommonItemStyleContent
public var normal: Style?
public var emphasis: Style?
public init() { }
}
public typealias EmphasisItemStyle = ItemStyle
extension ItemStyle: Enumable {
public enum Enums {
case normal(Style), emphasis(Style)
}
public typealias ContentEnum = Enums
public convenience init(_ elements: Enums...) {
self.init()
for ele in elements {
switch ele {
case let .normal(normal):
self.normal = normal
case let .emphasis(emphasis):
self.emphasis = emphasis
}
}
}
}
extension ItemStyle: Mappable {
public func mapping(_ map: Mapper) {
map["normal"] = normal
map["emphasis"] = emphasis
}
}
| mit | 95a503fbfa1df709659384807e5859e1 | 29.736842 | 264 | 0.601027 | 4.80376 | false | false | false | false |
ProcedureKit/ProcedureKit | Sources/ProcedureKit/Support.swift | 2 | 5103 | //
// ProcedureKit
//
// Copyright © 2015-2018 ProcedureKit. All rights reserved.
//
import Foundation
import Dispatch
internal func _abstractMethod(file: StaticString = #file, line: UInt = #line) {
fatalError("Method must be overriden", file: file, line: line)
}
extension Dictionary {
internal init<S: Sequence>(sequence: S, keyMapper: (Value) -> Key?) where S.Iterator.Element == Value {
self.init()
for item in sequence {
if let key = keyMapper(item) {
self[key] = item
}
}
}
}
// MARK: - Thread Safety
protocol ReadWriteLock {
mutating func read<T>(_ block: () throws -> T) rethrows -> T
mutating func write_async(_ block: @escaping () -> Void, completion: (() -> Void)?)
mutating func write_sync<T>(_ block: () throws -> T) rethrows -> T
}
extension ReadWriteLock {
mutating func write_async(_ block: @escaping () -> Void) {
write_async(block, completion: nil)
}
}
struct Lock: ReadWriteLock {
let queue = DispatchQueue.concurrent(label: "run.kit.procedure.ProcedureKit.Lock", qos: .userInitiated)
mutating func read<T>(_ block: () throws -> T) rethrows -> T {
return try queue.sync(execute: block)
}
mutating func write_async(_ block: @escaping () -> Void, completion: (() -> Void)?) {
queue.async(group: nil, flags: [.barrier]) {
block()
if let completion = completion {
DispatchQueue.main.async(execute: completion)
}
}
}
mutating func write_sync<T>(_ block: () throws -> T) rethrows -> T {
let result = try queue.sync(flags: [.barrier]) {
try block()
}
return result
}
}
/// A wrapper class for a pthread_mutex
final public class PThreadMutex {
private var mutex = pthread_mutex_t()
public init() {
let result = pthread_mutex_init(&mutex, nil)
precondition(result == 0, "Failed to create pthread mutex")
}
deinit {
let result = pthread_mutex_destroy(&mutex)
assert(result == 0, "Failed to destroy mutex")
}
fileprivate func lock() {
let result = pthread_mutex_lock(&mutex)
assert(result == 0, "Failed to lock mutex")
}
fileprivate func unlock() {
let result = pthread_mutex_unlock(&mutex)
assert(result == 0, "Failed to unlock mutex")
}
/// Convenience API to execute block after acquiring the lock
///
/// - Parameter block: the block to run
/// - Returns: returns the return value of the block
public func withCriticalScope<T>(block: () -> T) -> T {
lock()
defer { unlock() }
let value = block()
return value
}
}
public class Protector<T> {
private var lock = PThreadMutex()
private var ward: T
public init(_ ward: T) {
self.ward = ward
}
public var access: T {
var value: T?
lock.lock()
value = ward
lock.unlock()
return value!
}
public func read<U>(_ block: (T) -> U) -> U {
return lock.withCriticalScope { block(self.ward) }
}
/// Synchronously modify the protected value
///
/// - Returns: The value returned by the `block`, if any. (discardable)
@discardableResult public func write<U>(_ block: (inout T) -> U) -> U {
return lock.withCriticalScope { block(&self.ward) }
}
/// Synchronously overwrite the protected value
public func overwrite(with newValue: T) {
write { (ward: inout T) in ward = newValue }
}
}
public extension Protector where T: RangeReplaceableCollection {
func append(_ newElement: T.Iterator.Element) {
write { (ward: inout T) in
ward.append(newElement)
}
}
func append<S: Sequence>(contentsOf newElements: S) where S.Iterator.Element == T.Iterator.Element {
write { (ward: inout T) in
ward.append(contentsOf: newElements)
}
}
func append<C: Collection>(contentsOf newElements: C) where C.Iterator.Element == T.Iterator.Element {
write { (ward: inout T) in
ward.append(contentsOf: newElements)
}
}
}
public extension Protector where T: Strideable {
func advance(by stride: T.Stride) {
write { (ward: inout T) in
ward = ward.advanced(by: stride)
}
}
}
public extension NSLock {
/// Convenience API to execute block after acquiring the lock
///
/// - Parameter block: the block to run
/// - Returns: returns the return value of the block
func withCriticalScope<T>(block: () -> T) -> T {
lock()
let value = block()
unlock()
return value
}
}
public extension NSRecursiveLock {
/// Convenience API to execute block after acquiring the lock
///
/// - Parameter block: the block to run
/// - Returns: returns the return value of the block
func withCriticalScope<T>(block: () -> T) -> T {
lock()
let value = block()
unlock()
return value
}
}
| mit | cc3f1e2ef98b779a714fede6239cb71c | 25.572917 | 107 | 0.588397 | 4.185398 | false | false | false | false |
SuperAwesomeLTD/sa-mobile-sdk-ios | Adapters/AdMob/Example/SuperAwesomeAdMob/AdMobViewController.swift | 1 | 5410 | //
// ViewController.swift
// SuperAwesomeAdMob
//
// Created by Gunhan Sancar on 06/02/2020.
// Copyright (c) 2020 Gunhan Sancar. All rights reserved.
//
import UIKit
import SuperAwesome
import SuperAwesomeAdMob
import GoogleMobileAds
class AdMobViewController: UIViewController {
private var bannerAdUnitId = "ca-app-pub-2222631699890286/4083046906"
private var interstitialAdUnitId = "ca-app-pub-2222631699890286/6406350858"
private var rewardAdUnitId = "ca-app-pub-2222631699890286/3695609060"
private var bannerView: GADBannerView!
private var interstitial: GADInterstitialAd?
private var rewardedAd: GADRewardedAd?
override func viewDidLoad() {
super.viewDidLoad()
// Banner Ad
createAndLoadBannerAd()
// Intestitial Ad
createAndLoadInterstitial()
// Rewarded Ad
createAndLoadRewardedAd()
}
func createAndLoadBannerAd() {
let extra = SAAdMobCustomEventExtra()
extra.testEnabled = false
extra.parentalGateEnabled = false
extra.trasparentEnabled = true
let extras = GADCustomEventExtras()
extras.setExtras(extra as? [AnyHashable: Any], forLabel: "iOSBannerCustomEvent")
let request = GADRequest()
request.register(extras)
bannerView = GADBannerView(adSize: kGADAdSizeBanner)
bannerView.translatesAutoresizingMaskIntoConstraints = false
view.addSubview(bannerView)
view.addConstraints([
NSLayoutConstraint(item: bannerView, attribute: .top,
relatedBy: .equal, toItem: topLayoutGuide,
attribute: .bottom, multiplier: 1, constant: 0),
NSLayoutConstraint(item: bannerView, attribute: .centerX,
relatedBy: .equal, toItem: view,
attribute: .centerX, multiplier: 1, constant: 0)
])
bannerView.adUnitID = bannerAdUnitId
bannerView.rootViewController = self
bannerView.delegate = self
bannerView.load(request)
}
func createAndLoadInterstitial() {
GADInterstitialAd.load(withAdUnitID: interstitialAdUnitId, request: nil) { interstitial, error in
if error != nil {
print("[SuperAwesome | AdMob] interstitial failed to load case")
} else {
print("[SuperAwesome | AdMob] interstitial successfully loaded")
print("[SuperAwesome | AdMob] interstitial adapter class name: \(interstitial?.responseInfo.adNetworkClassName ?? "")")
}
self.interstitial = interstitial
}
}
func createAndLoadRewardedAd() {
let extra = SAAdMobVideoExtra()
extra.testEnabled = false
extra.closeAtEndEnabled = true
extra.closeButtonEnabled = true
extra.parentalGateEnabled = true
extra.smallCLickEnabled = true
// extra.configuration = STAGING;
// extra.orientation = LANDSCAPE;
let request = GADRequest()
request.register(extra)
GADRewardedAd.load(withAdUnitID: rewardAdUnitId, request: request) { rewardedAd, error in
if error != nil {
print("[SuperAwesome | AdMob] RewardedAd failed to load case")
} else {
print("[SuperAwesome | AdMob] RewardedAd successfully loaded")
print("[SuperAwesome | AdMob] RewardedAd adapter class name: \(rewardedAd?.responseInfo.adNetworkClassName ?? "")")
}
self.rewardedAd = rewardedAd
}
}
func addBannerView(bannerView: UIView?) {
guard let bannerView = bannerView else { return }
bannerView.translatesAutoresizingMaskIntoConstraints = false
view.addSubview(bannerView)
view.addConstraints([
NSLayoutConstraint(item: bannerView,
attribute: .top,
relatedBy: .equal,
toItem: topLayoutGuide,
attribute: .bottom,
multiplier: 1,
constant: 0),
NSLayoutConstraint(item: bannerView,
attribute: .centerX,
relatedBy: .equal,
toItem: view,
attribute: .centerX,
multiplier: 1,
constant: 0)
])
}
@IBAction func showInterstitial(_ sender: Any) {
if let ad = interstitial {
ad.present(fromRootViewController: self)
} else {
print("Ad wasn't ready")
}
}
@IBAction func showVideo(_ sender: Any) {
if let ad = rewardedAd {
ad.present(fromRootViewController: self) {
}
} else {
print("[SuperAwesome | AdMob] Video ad wasn't ready")
}
}
}
extension AdMobViewController: GADBannerViewDelegate {
func adViewDidReceiveAd(_ bannerView: GADBannerView) {
print("[SuperAwesome | AdMob] adView:adViewDidReceiveAd:")
}
func bannerViewDidDismissScreen(_ bannerView: GADBannerView) {
print("[SuperAwesome | AdMob] adView:adViewDidDismissScreen:")
}
}
extension AdMobViewController: GADFullScreenContentDelegate {
}
| lgpl-3.0 | 4aa2db615e49fa7c2dc1e7f920cecf17 | 33.903226 | 135 | 0.596118 | 5.404595 | false | false | false | false |
MacToolbox/IP | IP/AppDelegate.swift | 1 | 1100 | //
// AppDelegate.swift
// IP
//
// Created by Dmytro Morozov on 05/09/2016.
// Copyright © 2016 Dmytro Morozov. All rights reserved.
//
import Cocoa
@NSApplicationMain
class AppDelegate: NSObject, NSApplicationDelegate {
fileprivate let statusItem = NSStatusBar.system().statusItem(withLength: NSVariableStatusItemLength)
@IBOutlet fileprivate weak var menu: NSMenu!
func applicationDidFinishLaunching(_ aNotification: Notification) {
statusItem.menu = menu
let addresses = Utility.getAddresses(.v4, excludeLocal: true)
if let address = addresses.first {
statusItem.button?.title = address
}
}
@IBAction func copy(_ sender: AnyObject) {
if let address = statusItem.button?.title {
let pasteboard = NSPasteboard.general()
pasteboard.declareTypes([NSPasteboardTypeString], owner: nil)
pasteboard.setString(address, forType: NSPasteboardTypeString)
}
}
@IBAction fileprivate func quit(_ sender: AnyObject) {
NSApp.terminate(sender)
}
}
| mit | 333c842ca7466eed9e9b6abe22977e29 | 27.179487 | 104 | 0.66697 | 4.862832 | false | false | false | false |
guarani/Collapsible-UINavigationBar | TestShrinkingNavBar/UINavigationController+CollapsableNavigationBar.swift | 2 | 3305 | //
// PVSCollapsibleNavigationController.swift
// TestBarButtonItems
//
// Created by Paul Von Schrottky on 4/3/15.
// Copyright (c) 2015 Paul Von Schrottky. All rights reserved.
//
import UIKit
// These are the variables that configure the navigation bar.
// Change these to suit your needs.
private var defaultNavigationBarHeight: CGFloat = 44.0
private var currentNavigationBarHeight = defaultNavigationBarHeight
private let defaultNavigationBarTitleFontSize: CGFloat = 20.0
private let shrinkBy: CGFloat = 25.0 // The amount that the navigation bar height shrinks.
// Other globals.
private let customTitleLabel = UILabel()
private var navBarTitleHeightConstraint: NSLayoutConstraint!
extension UINavigationBar {
public override func sizeThatFits(size: CGSize) -> CGSize {
return CGSizeMake(self.superview!.frame.size.width, currentNavigationBarHeight)
}
}
extension UINavigationController {
func pvs_collapsibleBar() {
self.topViewController.navigationItem.titleView = customTitleLabel
customTitleLabel.font = UIFont.boldSystemFontOfSize(20)
customTitleLabel.text = "Hello, mundo!"
customTitleLabel.setTranslatesAutoresizingMaskIntoConstraints(false)
self.view.addConstraint(NSLayoutConstraint(item: customTitleLabel, attribute: .CenterX, relatedBy: .Equal, toItem: self.view, attribute: .CenterX, multiplier: 1.0, constant: 0))
self.view.addConstraint(NSLayoutConstraint(item: customTitleLabel, attribute: .CenterY, relatedBy: .Equal, toItem: self.navigationBar, attribute: .CenterY, multiplier: 1.0, constant: 0))
}
func pvs_collapisbleBarScrollViewDidScroll(scrollView: UIScrollView) {
let statusBarSize = UIApplication.sharedApplication().statusBarFrame.size
let statusBarHeight = min(statusBarSize.width, statusBarSize.height)
var yOffset = scrollView.contentOffset.y
if self.topViewController.automaticallyAdjustsScrollViewInsets {
yOffset += statusBarHeight
yOffset += self.navigationBar.frame.size.height
}
println("yOffset: \(yOffset)")
if yOffset < 0 {
customTitleLabel.font = customTitleLabel.font.fontWithSize(defaultNavigationBarTitleFontSize)
currentNavigationBarHeight = defaultNavigationBarHeight
self.navigationBar.tintColor = self.navigationBar.tintColor.colorWithAlphaComponent(1)
} else if yOffset < shrinkBy {
currentNavigationBarHeight = defaultNavigationBarHeight - yOffset
customTitleLabel.font = customTitleLabel.font.fontWithSize(defaultNavigationBarTitleFontSize - (yOffset / 3))
self.navigationBar.tintColor = self.navigationBar.tintColor.colorWithAlphaComponent(1 - (yOffset / shrinkBy))
} else {
currentNavigationBarHeight = defaultNavigationBarHeight - shrinkBy
customTitleLabel.font = customTitleLabel.font.fontWithSize(defaultNavigationBarTitleFontSize - (shrinkBy / 3))
self.navigationBar.tintColor = self.navigationBar.tintColor.colorWithAlphaComponent(0)
}
if let constraint = navBarTitleHeightConstraint {
constraint.constant = CGFloat(currentNavigationBarHeight)
}
self.navigationBar.sizeToFit()
}
}
| mit | 01f3d18d209db4ef02e7d8415f40ea1a | 47.602941 | 194 | 0.73646 | 5.480929 | false | false | false | false |
apple/swift-corelibs-foundation | Sources/Foundation/CGFloat.swift | 1 | 26182 | // This source file is part of the Swift.org open source project
//
// Copyright (c) 2014 - 2016, 2018 Apple Inc. and the Swift project authors
// Licensed under Apache License v2.0 with Runtime Library Exception
//
// See http://swift.org/LICENSE.txt for license information
// See http://swift.org/CONTRIBUTORS.txt for the list of Swift project authors
//
@frozen
public struct CGFloat {
#if arch(i386) || arch(arm) || arch(wasm32)
/// The native type used to store the CGFloat, which is Float on
/// 32-bit architectures and Double on 64-bit architectures.
public typealias NativeType = Float
#elseif arch(x86_64) || arch(arm64) || arch(s390x) || arch(powerpc64) || arch(powerpc64le)
/// The native type used to store the CGFloat, which is Float on
/// 32-bit architectures and Double on 64-bit architectures.
public typealias NativeType = Double
#else
#error("This architecture isn't known. Add it to the 32-bit or 64-bit line.")
#endif
@_transparent public init() {
self.native = 0.0
}
@_transparent public init(_ value: Float) {
self.native = NativeType(value)
}
@_transparent public init(_ value: Double) {
self.native = NativeType(value)
}
#if !(os(Windows) || os(Android)) && (arch(i386) || arch(x86_64))
@_transparent public init(_ value: Float80) {
self.native = NativeType(value)
}
#endif
@_transparent public init(_ value: CGFloat) {
self.native = value.native
}
/// Creates a new value, rounded to the closest possible representation.
///
/// If two representable values are equally close, the result is the value
/// with more trailing zeros in its significand bit pattern.
///
/// - Parameter value: The integer to convert to a floating-point value.
public init(_ value: UInt8) {
self.native = NativeType(value)
}
/// Creates a new value, rounded to the closest possible representation.
///
/// If two representable values are equally close, the result is the value
/// with more trailing zeros in its significand bit pattern.
///
/// - Parameter value: The integer to convert to a floating-point value.
public init(_ value: Int8) {
self.native = NativeType(value)
}
/// Creates a new value, rounded to the closest possible representation.
///
/// If two representable values are equally close, the result is the value
/// with more trailing zeros in its significand bit pattern.
///
/// - Parameter value: The integer to convert to a floating-point value.
public init(_ value: UInt16) {
self.native = NativeType(value)
}
/// Creates a new value, rounded to the closest possible representation.
///
/// If two representable values are equally close, the result is the value
/// with more trailing zeros in its significand bit pattern.
///
/// - Parameter value: The integer to convert to a floating-point value.
public init(_ value: Int16) {
self.native = NativeType(value)
}
/// Creates a new value, rounded to the closest possible representation.
///
/// If two representable values are equally close, the result is the value
/// with more trailing zeros in its significand bit pattern.
///
/// - Parameter value: The integer to convert to a floating-point value.
public init(_ value: UInt32) {
self.native = NativeType(value)
}
/// Creates a new value, rounded to the closest possible representation.
///
/// If two representable values are equally close, the result is the value
/// with more trailing zeros in its significand bit pattern.
///
/// - Parameter value: The integer to convert to a floating-point value.
public init(_ value: Int32) {
self.native = NativeType(value)
}
/// Creates a new value, rounded to the closest possible representation.
///
/// If two representable values are equally close, the result is the value
/// with more trailing zeros in its significand bit pattern.
///
/// - Parameter value: The integer to convert to a floating-point value.
public init(_ value: UInt64) {
self.native = NativeType(value)
}
/// Creates a new value, rounded to the closest possible representation.
///
/// If two representable values are equally close, the result is the value
/// with more trailing zeros in its significand bit pattern.
///
/// - Parameter value: The integer to convert to a floating-point value.
public init(_ value: Int64) {
self.native = NativeType(value)
}
/// Creates a new value, rounded to the closest possible representation.
///
/// If two representable values are equally close, the result is the value
/// with more trailing zeros in its significand bit pattern.
///
/// - Parameter value: The integer to convert to a floating-point value.
public init(_ value: UInt) {
self.native = NativeType(value)
}
/// Creates a new value, rounded to the closest possible representation.
///
/// If two representable values are equally close, the result is the value
/// with more trailing zeros in its significand bit pattern.
///
/// - Parameter value: The integer to convert to a floating-point value.
public init(_ value: Int) {
self.native = NativeType(value)
}
/// The native value.
public var native: NativeType
}
extension CGFloat : SignedNumeric {
// FIXME(integers): implement
public init?<T : BinaryInteger>(exactly source: T) {
fatalError()
}
@_transparent
public var magnitude: CGFloat {
return CGFloat(Swift.abs(native))
}
}
extension CGFloat : BinaryFloatingPoint {
public typealias RawSignificand = UInt
public typealias Exponent = Int
@_transparent
public static var exponentBitCount: Int {
return NativeType.exponentBitCount
}
@_transparent
public static var significandBitCount: Int {
return NativeType.significandBitCount
}
// Conversions to/from integer encoding. These are not part of the
// BinaryFloatingPoint prototype because there's no guarantee that an
// integer type of the same size actually exists (e.g. Float80).
@_transparent
public var bitPattern: UInt {
return UInt(native.bitPattern)
}
@_transparent
public init(bitPattern: UInt) {
#if arch(i386) || arch(arm) || arch(wasm32)
native = NativeType(bitPattern: UInt32(bitPattern))
#elseif arch(x86_64) || arch(arm64) || arch(s390x) || arch(powerpc64) || arch(powerpc64le)
native = NativeType(bitPattern: UInt64(bitPattern))
#else
#error("This architecture isn't known. Add it to the 32-bit or 64-bit line.")
#endif
}
@_transparent
public var sign: FloatingPointSign {
return native.sign
}
@_transparent
public var exponentBitPattern: UInt {
return native.exponentBitPattern
}
@_transparent
public var significandBitPattern: UInt {
return UInt(native.significandBitPattern)
}
@_transparent
public init(sign: FloatingPointSign,
exponentBitPattern: UInt,
significandBitPattern: UInt) {
native = NativeType(sign: sign,
exponentBitPattern: exponentBitPattern,
significandBitPattern: NativeType.RawSignificand(significandBitPattern))
}
@_transparent
public init(nan payload: RawSignificand, signaling: Bool) {
native = NativeType(nan: NativeType.RawSignificand(payload),
signaling: signaling)
}
@_transparent
public static var infinity: CGFloat {
return CGFloat(NativeType.infinity)
}
@_transparent
public static var nan: CGFloat {
return CGFloat(NativeType.nan)
}
@_transparent
public static var signalingNaN: CGFloat {
return CGFloat(NativeType.signalingNaN)
}
@available(*, unavailable, renamed: "nan")
public static var quietNaN: CGFloat {
fatalError("unavailable")
}
@_transparent
public static var greatestFiniteMagnitude: CGFloat {
return CGFloat(NativeType.greatestFiniteMagnitude)
}
@_transparent
public static var pi: CGFloat {
return CGFloat(NativeType.pi)
}
@_transparent
public var ulp: CGFloat {
return CGFloat(native.ulp)
}
@_transparent
public static var leastNormalMagnitude: CGFloat {
return CGFloat(NativeType.leastNormalMagnitude)
}
@_transparent
public static var leastNonzeroMagnitude: CGFloat {
return CGFloat(NativeType.leastNonzeroMagnitude)
}
@_transparent
public var exponent: Int {
return native.exponent
}
@_transparent
public var significand: CGFloat {
return CGFloat(native.significand)
}
@_transparent
public init(sign: FloatingPointSign, exponent: Int, significand: CGFloat) {
native = NativeType(sign: sign,
exponent: exponent, significand: significand.native)
}
@_transparent
public mutating func round(_ rule: FloatingPointRoundingRule) {
native.round(rule)
}
@_transparent
public var nextUp: CGFloat {
return CGFloat(native.nextUp)
}
@_transparent
public mutating func negate() {
native.negate()
}
@_transparent
public static func +=(_ lhs: inout CGFloat, _ rhs: CGFloat) {
lhs.native += rhs.native
}
@_transparent
public static func -=(_ lhs: inout CGFloat, _ rhs: CGFloat) {
lhs.native -= rhs.native
}
@_transparent
public static func *=(_ lhs: inout CGFloat, _ rhs: CGFloat) {
lhs.native *= rhs.native
}
@_transparent
public static func /=(_ lhs: inout CGFloat, _ rhs: CGFloat) {
lhs.native /= rhs.native
}
@_transparent
public mutating func formTruncatingRemainder(dividingBy other: CGFloat) {
native.formTruncatingRemainder(dividingBy: other.native)
}
@_transparent
public mutating func formRemainder(dividingBy other: CGFloat) {
native.formRemainder(dividingBy: other.native)
}
@_transparent
public mutating func formSquareRoot( ) {
native.formSquareRoot( )
}
@_transparent
public mutating func addProduct(_ lhs: CGFloat, _ rhs: CGFloat) {
native.addProduct(lhs.native, rhs.native)
}
@_transparent
public func isEqual(to other: CGFloat) -> Bool {
return self.native.isEqual(to: other.native)
}
@_transparent
public func isLess(than other: CGFloat) -> Bool {
return self.native.isLess(than: other.native)
}
@_transparent
public func isLessThanOrEqualTo(_ other: CGFloat) -> Bool {
return self.native.isLessThanOrEqualTo(other.native)
}
@_transparent
public var isNormal: Bool {
return native.isNormal
}
@_transparent
public var isFinite: Bool {
return native.isFinite
}
@_transparent
public var isZero: Bool {
return native.isZero
}
@_transparent
public var isSubnormal: Bool {
return native.isSubnormal
}
@_transparent
public var isInfinite: Bool {
return native.isInfinite
}
@_transparent
public var isNaN: Bool {
return native.isNaN
}
@_transparent
public var isSignalingNaN: Bool {
return native.isSignalingNaN
}
@available(*, unavailable, renamed: "isSignalingNaN")
public var isSignaling: Bool {
fatalError("unavailable")
}
@_transparent
public var isCanonical: Bool {
return true
}
@_transparent
public var floatingPointClass: FloatingPointClassification {
return native.floatingPointClass
}
@_transparent
public var binade: CGFloat {
return CGFloat(native.binade)
}
@_transparent
public var significandWidth: Int {
return native.significandWidth
}
/// Create an instance initialized to `value`.
@_transparent
public init(floatLiteral value: NativeType) {
native = value
}
/// Create an instance initialized to `value`.
@_transparent
public init(integerLiteral value: Int) {
native = NativeType(value)
}
}
extension CGFloat {
@available(*, unavailable, renamed: "leastNormalMagnitude")
public static var min: CGFloat {
fatalError("unavailable")
}
@available(*, unavailable, renamed: "greatestFiniteMagnitude")
public static var max: CGFloat {
fatalError("unavailable")
}
@available(*, unavailable, message: "Please use the `abs(_:)` free function")
public static func abs(_ x: CGFloat) -> CGFloat {
fatalError("unavailable")
}
}
@available(*, unavailable, renamed: "CGFloat.leastNormalMagnitude")
public var CGFLOAT_MIN: CGFloat {
fatalError("unavailable")
}
@available(*, unavailable, renamed: "CGFloat.greatestFiniteMagnitude")
public var CGFLOAT_MAX: CGFloat {
fatalError("unavailable")
}
extension CGFloat : CustomReflectable {
/// Returns a mirror that reflects `self`.
public var customMirror: Mirror {
return Mirror(reflecting: native)
}
}
extension CGFloat : CustomStringConvertible {
/// A textual representation of `self`.
public var description: String {
return native.description
}
}
extension CGFloat : Hashable {
/// The hash value.
///
/// **Axiom:** `x == y` implies `x.hashValue == y.hashValue`
///
/// - Note: the hash value is not guaranteed to be stable across
/// different invocations of the same program. Do not persist the
/// hash value across program runs.
@_transparent
public var hashValue: Int {
return native.hashValue
}
@_transparent
public func hash(into hasher: inout Hasher) {
hasher.combine(native)
}
@_transparent
public func _rawHashValue(seed: Int) -> Int {
return native._rawHashValue(seed: seed)
}
}
extension UInt8 {
@_transparent
public init(_ value: CGFloat) {
self = UInt8(value.native)
}
}
extension Int8 {
@_transparent
public init(_ value: CGFloat) {
self = Int8(value.native)
}
}
extension UInt16 {
@_transparent
public init(_ value: CGFloat) {
self = UInt16(value.native)
}
}
extension Int16 {
@_transparent
public init(_ value: CGFloat) {
self = Int16(value.native)
}
}
extension UInt32 {
@_transparent
public init(_ value: CGFloat) {
self = UInt32(value.native)
}
}
extension Int32 {
@_transparent
public init(_ value: CGFloat) {
self = Int32(value.native)
}
}
extension UInt64 {
@_transparent
public init(_ value: CGFloat) {
self = UInt64(value.native)
}
}
extension Int64 {
@_transparent
public init(_ value: CGFloat) {
self = Int64(value.native)
}
}
extension UInt {
@_transparent
public init(_ value: CGFloat) {
self = UInt(value.native)
}
}
extension Int {
@_transparent
public init(_ value: CGFloat) {
self = Int(value.native)
}
}
extension Double {
@_transparent
public init(_ value: CGFloat) {
self = Double(value.native)
}
}
extension Float {
@_transparent
public init(_ value: CGFloat) {
self = Float(value.native)
}
}
//===----------------------------------------------------------------------===//
// Standard Operator Table
//===----------------------------------------------------------------------===//
// TODO: These should not be necessary, since they're already provided by
// <T: FloatingPoint>, but in practice they are currently needed to
// disambiguate overloads. We should find a way to remove them, either by
// tweaking the overload resolution rules, or by removing the other
// definitions in the standard lib, or both.
extension CGFloat {
@_transparent
public static func +(lhs: CGFloat, rhs: CGFloat) -> CGFloat {
var lhs = lhs
lhs += rhs
return lhs
}
@_transparent
public static func -(lhs: CGFloat, rhs: CGFloat) -> CGFloat {
var lhs = lhs
lhs -= rhs
return lhs
}
@_transparent
public static func *(lhs: CGFloat, rhs: CGFloat) -> CGFloat {
var lhs = lhs
lhs *= rhs
return lhs
}
@_transparent
public static func /(lhs: CGFloat, rhs: CGFloat) -> CGFloat {
var lhs = lhs
lhs /= rhs
return lhs
}
}
//===----------------------------------------------------------------------===//
// Strideable Conformance
//===----------------------------------------------------------------------===//
extension CGFloat : Strideable {
/// Returns a stride `x` such that `self.advanced(by: x)` approximates
/// `other`.
///
/// - Complexity: O(1).
@_transparent
public func distance(to other: CGFloat) -> CGFloat {
return CGFloat(other.native - self.native)
}
/// Returns a `Self` `x` such that `self.distance(to: x)` approximates
/// `n`.
///
/// - Complexity: O(1).
@_transparent
public func advanced(by amount: CGFloat) -> CGFloat {
return CGFloat(self.native + amount.native)
}
}
//===----------------------------------------------------------------------===//
// Deprecated operators
//===----------------------------------------------------------------------===//
@available(*, unavailable, message: "Use truncatingRemainder instead")
public func %(lhs: CGFloat, rhs: CGFloat) -> CGFloat {
fatalError("% is not available.")
}
@available(*, unavailable, message: "Use formTruncatingRemainder instead")
public func %=(lhs: inout CGFloat, rhs: CGFloat) {
fatalError("%= is not available.")
}
//===----------------------------------------------------------------------===//
// tgmath
//===----------------------------------------------------------------------===//
@_transparent
public func acos(_ x: CGFloat) -> CGFloat {
return CGFloat(acos(x.native))
}
@_transparent
public func cos(_ x: CGFloat) -> CGFloat {
return CGFloat(cos(x.native))
}
@_transparent
public func sin(_ x: CGFloat) -> CGFloat {
return CGFloat(sin(x.native))
}
@_transparent
public func asin(_ x: CGFloat) -> CGFloat {
return CGFloat(asin(x.native))
}
@_transparent
public func atan(_ x: CGFloat) -> CGFloat {
return CGFloat(atan(x.native))
}
@_transparent
public func tan(_ x: CGFloat) -> CGFloat {
return CGFloat(tan(x.native))
}
@_transparent
public func acosh(_ x: CGFloat) -> CGFloat {
return CGFloat(acosh(x.native))
}
@_transparent
public func asinh(_ x: CGFloat) -> CGFloat {
return CGFloat(asinh(x.native))
}
@_transparent
public func atanh(_ x: CGFloat) -> CGFloat {
return CGFloat(atanh(x.native))
}
@_transparent
public func cosh(_ x: CGFloat) -> CGFloat {
return CGFloat(cosh(x.native))
}
@_transparent
public func sinh(_ x: CGFloat) -> CGFloat {
return CGFloat(sinh(x.native))
}
@_transparent
public func tanh(_ x: CGFloat) -> CGFloat {
return CGFloat(tanh(x.native))
}
@_transparent
public func exp(_ x: CGFloat) -> CGFloat {
return CGFloat(exp(x.native))
}
@_transparent
public func exp2(_ x: CGFloat) -> CGFloat {
return CGFloat(exp2(x.native))
}
@_transparent
public func expm1(_ x: CGFloat) -> CGFloat {
return CGFloat(expm1(x.native))
}
@_transparent
public func log(_ x: CGFloat) -> CGFloat {
return CGFloat(log(x.native))
}
@_transparent
public func log10(_ x: CGFloat) -> CGFloat {
return CGFloat(log10(x.native))
}
@_transparent
public func log2(_ x: CGFloat) -> CGFloat {
return CGFloat(log2(x.native))
}
@_transparent
public func log1p(_ x: CGFloat) -> CGFloat {
return CGFloat(log1p(x.native))
}
@_transparent
public func logb(_ x: CGFloat) -> CGFloat {
return CGFloat(logb(x.native))
}
@_transparent
public func cbrt(_ x: CGFloat) -> CGFloat {
return CGFloat(cbrt(x.native))
}
@_transparent
public func erf(_ x: CGFloat) -> CGFloat {
return CGFloat(erf(x.native))
}
@_transparent
public func erfc(_ x: CGFloat) -> CGFloat {
return CGFloat(erfc(x.native))
}
@_transparent
public func tgamma(_ x: CGFloat) -> CGFloat {
return CGFloat(tgamma(x.native))
}
@_transparent
public func nearbyint(_ x: CGFloat) -> CGFloat {
return CGFloat(x.native.rounded(.toNearestOrEven))
}
@_transparent
public func rint(_ x: CGFloat) -> CGFloat {
return CGFloat(x.native.rounded(.toNearestOrEven))
}
@_transparent
public func atan2(_ lhs: CGFloat, _ rhs: CGFloat) -> CGFloat {
return CGFloat(atan2(lhs.native, rhs.native))
}
@_transparent
public func hypot(_ lhs: CGFloat, _ rhs: CGFloat) -> CGFloat {
return CGFloat(hypot(lhs.native, rhs.native))
}
@_transparent
public func pow(_ lhs: CGFloat, _ rhs: CGFloat) -> CGFloat {
return CGFloat(pow(lhs.native, rhs.native))
}
@_transparent
public func copysign(_ lhs: CGFloat, _ rhs: CGFloat) -> CGFloat {
return CGFloat(copysign(lhs.native, rhs.native))
}
@_transparent
public func nextafter(_ lhs: CGFloat, _ rhs: CGFloat) -> CGFloat {
return CGFloat(nextafter(lhs.native, rhs.native))
}
@_transparent
public func fdim(_ lhs: CGFloat, _ rhs: CGFloat) -> CGFloat {
return CGFloat(fdim(lhs.native, rhs.native))
}
@_transparent
public func fmax(_ lhs: CGFloat, _ rhs: CGFloat) -> CGFloat {
return CGFloat(fmax(lhs.native, rhs.native))
}
@_transparent
public func fmin(_ lhs: CGFloat, _ rhs: CGFloat) -> CGFloat {
return CGFloat(fmin(lhs.native, rhs.native))
}
@_transparent
@available(*, unavailable, message: "use the floatingPointClass property.")
public func fpclassify(_ x: CGFloat) -> Int {
fatalError("unavailable")
}
@available(*, unavailable, message: "use the isNormal property.")
public func isnormal(_ value: CGFloat) -> Bool { return value.isNormal }
@available(*, unavailable, message: "use the isFinite property.")
public func isfinite(_ value: CGFloat) -> Bool { return value.isFinite }
@available(*, unavailable, message: "use the isInfinite property.")
public func isinf(_ value: CGFloat) -> Bool { return value.isInfinite }
@available(*, unavailable, message: "use the isNaN property.")
public func isnan(_ value: CGFloat) -> Bool { return value.isNaN }
@available(*, unavailable, message: "use the sign property.")
public func signbit(_ value: CGFloat) -> Int { return value.sign.rawValue }
@_transparent
public func modf(_ x: CGFloat) -> (CGFloat, CGFloat) {
let (ipart, fpart) = modf(x.native)
return (CGFloat(ipart), CGFloat(fpart))
}
@_transparent
public func ldexp(_ x: CGFloat, _ n: Int) -> CGFloat {
return CGFloat(scalbn(x.native, n))
}
@_transparent
public func frexp(_ x: CGFloat) -> (CGFloat, Int) {
let (frac, exp) = frexp(x.native)
return (CGFloat(frac), exp)
}
@_transparent
public func ilogb(_ x: CGFloat) -> Int {
return x.native.exponent
}
@_transparent
public func scalbn(_ x: CGFloat, _ n: Int) -> CGFloat {
return CGFloat(scalbn(x.native, n))
}
#if !os(Windows)
@_transparent
public func lgamma(_ x: CGFloat) -> (CGFloat, Int) {
let (value, sign) = lgamma(x.native)
return (CGFloat(value), sign)
}
#endif
@_transparent
public func remquo(_ x: CGFloat, _ y: CGFloat) -> (CGFloat, Int) {
let (rem, quo) = remquo(x.native, y.native)
return (CGFloat(rem), quo)
}
@_transparent
public func nan(_ tag: String) -> CGFloat {
return CGFloat(nan(tag) as CGFloat.NativeType)
}
@_transparent
public func j0(_ x: CGFloat) -> CGFloat {
return CGFloat(j0(Double(x.native)))
}
@_transparent
public func j1(_ x: CGFloat) -> CGFloat {
return CGFloat(j1(Double(x.native)))
}
@_transparent
public func jn(_ n: Int, _ x: CGFloat) -> CGFloat {
return CGFloat(jn(n, Double(x.native)))
}
@_transparent
public func y0(_ x: CGFloat) -> CGFloat {
return CGFloat(y0(Double(x.native)))
}
@_transparent
public func y1(_ x: CGFloat) -> CGFloat {
return CGFloat(y1(Double(x.native)))
}
@_transparent
public func yn(_ n: Int, _ x: CGFloat) -> CGFloat {
return CGFloat(yn(n, Double(x.native)))
}
extension CGFloat : _CVarArgPassedAsDouble, _CVarArgAligned {
/// Transform `self` into a series of machine words that can be
/// appropriately interpreted by C varargs
@_transparent
public var _cVarArgEncoding: [Int] {
return native._cVarArgEncoding
}
/// Return the required alignment in bytes of
/// the value returned by `_cVarArgEncoding`.
@_transparent
public var _cVarArgAlignment: Int {
return native._cVarArgAlignment
}
}
extension CGFloat : Codable {
@_transparent
public init(from decoder: Decoder) throws {
let container = try decoder.singleValueContainer()
do {
self.native = try container.decode(NativeType.self)
} catch DecodingError.typeMismatch(let type, let context) {
// We may have encoded as a different type on a different platform. A
// strict fixed-format decoder may disallow a conversion, so let's try the
// other type.
do {
if NativeType.self == Float.self {
self.native = NativeType(try container.decode(Double.self))
} else {
self.native = NativeType(try container.decode(Float.self))
}
} catch {
// Failed to decode as the other type, too. This is neither a Float nor
// a Double. Throw the old error; we don't want to clobber the original
// info.
throw DecodingError.typeMismatch(type, context)
}
}
}
@_transparent
public func encode(to encoder: Encoder) throws {
var container = encoder.singleValueContainer()
try container.encode(self.native)
}
}
| apache-2.0 | d5512c0b8a74ff1fc84e83923a159871 | 25.853333 | 100 | 0.627454 | 4.395165 | false | false | false | false |
TrustWallet/trust-wallet-ios | Trust/Extensions/UIAlertController.swift | 1 | 1838 | // Copyright DApps Platform Inc. All rights reserved.
import Foundation
import UIKit
import Result
extension UIAlertController {
static func askPassword(
title: String = "",
message: String = "",
completion: @escaping (Result<String, ConfirmationError>) -> Void
) -> UIAlertController {
let alertController = UIAlertController(
title: title,
message: message,
preferredStyle: .alert
)
alertController.addAction(UIAlertAction(title: NSLocalizedString("OK", value: "OK", comment: ""), style: .default, handler: { _ -> Void in
let textField = alertController.textFields![0] as UITextField
let password = textField.text ?? ""
completion(.success(password))
}))
alertController.addAction(UIAlertAction(title: R.string.localizable.cancel(), style: .cancel, handler: { _ in
completion(.failure(ConfirmationError.cancel))
}))
alertController.addTextField(configurationHandler: {(textField: UITextField!) -> Void in
textField.placeholder = NSLocalizedString("Password", value: "Password", comment: "")
textField.isSecureTextEntry = true
})
return alertController
}
static func alertController(
title: String? = .none,
message: String? = .none,
style: UIAlertControllerStyle,
in navigationController: NavigationController
) -> UIAlertController {
let alertController = UIAlertController(title: title, message: message, preferredStyle: style)
alertController.popoverPresentationController?.sourceView = navigationController.view
alertController.popoverPresentationController?.sourceRect = navigationController.view.centerRect
return alertController
}
}
| gpl-3.0 | 252c9095bae3ae0a0b0e6559617dd880 | 39.844444 | 146 | 0.664309 | 5.74375 | false | false | false | false |
yoichitgy/swipe | core/SwipeParser.swift | 1 | 16355 | //
// SwipeParser.swift
// Swipe
//
// Created by satoshi on 6/4/15.
// Copyright (c) 2015 Satoshi Nakajima. All rights reserved.
//
// http://stackoverflow.com/questions/23790143/how-do-i-set-uicolor-to-this-exact-shade-green-that-i-want/23790739#23790739
// dropFirst http://stackoverflow.com/questions/28445917/what-is-the-most-succinct-way-to-remove-the-first-character-from-a-string-in-swi/28446142#28446142
#if os(OSX)
import Cocoa
public typealias UIColor = NSColor
public typealias UIFont = NSFont
#else
import UIKit
#endif
import ImageIO
class SwipeParser {
//let color = NSRegularExpression(pattern: "#[0-9A-F]6", options: NSRegularExpressionOptions.CaseInsensitive, error: nil)
static let colorMap = [
"red":UIColor.red,
"black":UIColor.black,
"blue":UIColor.blue,
"white":UIColor.white,
"green":UIColor.green,
"yellow":UIColor.yellow,
"purple":UIColor.purple,
"gray":UIColor.gray,
"darkGray":UIColor.darkGray,
"lightGray":UIColor.lightGray,
"brown":UIColor.brown,
"orange":UIColor.orange,
"cyan":UIColor.cyan,
"magenta":UIColor.magenta
]
static let regexColor = try! NSRegularExpression(pattern: "^#[A-F0-9]*$", options: NSRegularExpression.Options.caseInsensitive)
static func parseColor(_ value:Any?, defaultColor:CGColor = UIColor.clear.cgColor) -> CGColor {
if value == nil {
return defaultColor
}
if let rgba = value as? [String: Any] {
var red:CGFloat = 0.0, blue:CGFloat = 0.0, green:CGFloat = 0.0
var alpha:CGFloat = 1.0
if let v = rgba["r"] as? CGFloat {
red = v
}
if let v = rgba["g"] as? CGFloat {
green = v
}
if let v = rgba["b"] as? CGFloat {
blue = v
}
if let v = rgba["a"] as? CGFloat {
alpha = v
}
return UIColor(red: red, green: green, blue: blue, alpha: alpha).cgColor
} else if let key = value as? String {
if let color = colorMap[key] {
return color.cgColor
} else {
let results = regexColor.matches(in: key, options: NSRegularExpression.MatchingOptions(), range: NSMakeRange(0, key.characters.count))
if results.count > 0 {
let hex = String(key.characters.dropFirst())
let cstr = hex.cString(using: String.Encoding.ascii)
let v = strtoll(cstr!, nil, 16)
//NSLog("SwipeParser hex=\(hex), \(value)")
var r = Int64(0), g = Int64(0), b = Int64(0), a = Int64(255)
switch(hex.characters.count) {
case 3:
r = v / 0x100 * 0x11
g = v / 0x10 % 0x10 * 0x11
b = v % 0x10 * 0x11
case 4:
r = v / 0x1000 * 0x11
g = v / 0x100 % 0x10 * 0x11
b = v / 0x10 % 0x10 * 0x11
a = v % 0x10 * 0x11
case 6:
r = v / 0x10000
g = v / 0x100
b = v
case 8:
r = v / 0x1000000
g = v / 0x10000
b = v / 0x100
a = v
default:
break;
}
return UIColor(red: CGFloat(r)/255, green: CGFloat(g%256)/255, blue: CGFloat(b%256)/255, alpha: CGFloat(a%256)/255).cgColor
}
return UIColor.red.cgColor
}
}
return UIColor.green.cgColor
}
static func transformedPath(_ path:CGPath, param:[String:Any]?, size:CGSize) -> CGPath? {
if let value = param {
var scale:CGSize?
if let s = value["scale"] as? CGFloat {
scale = CGSize(width: s, height: s)
}
if let scales = value["scale"] as? [CGFloat], scales.count == 2 {
scale = CGSize(width: scales[0], height: scales[1])
}
if let s = scale {
var xf = CGAffineTransform(translationX: size.width / 2, y: size.height / 2)
xf = xf.scaledBy(x: s.width, y: s.height)
xf = xf.translatedBy(x: -size.width / 2, y: -size.height / 2)
return path.copy(using: &xf)!
}
}
return nil
}
static func parseTransform(_ param:[String:Any]?, scaleX:CGFloat, scaleY:CGFloat, base:[String:Any]?, fSkipTranslate:Bool, fSkipScale:Bool) -> CATransform3D? {
if let p = param {
var value = p
var xf = CATransform3DIdentity
var hasValue = false
if let b = base {
for key in ["translate", "rotate", "scale"] {
if let v = b[key], value[key]==nil {
value[key] = v
}
}
}
if fSkipTranslate {
if let b = base,
let translate = b["translate"] as? [CGFloat], translate.count == 2{
xf = CATransform3DTranslate(xf, translate[0] * scaleX, translate[1] * scaleY, 0)
}
} else {
if let translate = value["translate"] as? [CGFloat] {
if translate.count == 2 {
xf = CATransform3DTranslate(xf, translate[0] * scaleX, translate[1] * scaleY, 0)
}
hasValue = true
}
}
if let depth = value["depth"] as? CGFloat {
xf = CATransform3DTranslate(xf, 0, 0, depth)
hasValue = true
}
if let rot = value["rotate"] as? CGFloat {
xf = CATransform3DRotate(xf, rot * CGFloat(M_PI / 180.0), 0, 0, 1)
hasValue = true
}
if let rots = value["rotate"] as? [CGFloat], rots.count == 3 {
let m = CGFloat(M_PI / 180.0)
xf = CATransform3DRotate(xf, rots[0] * m, 1, 0, 0)
xf = CATransform3DRotate(xf, rots[1] * m, 0, 1, 0)
xf = CATransform3DRotate(xf, rots[2] * m, 0, 0, 1)
hasValue = true
}
if !fSkipScale {
if let scale = value["scale"] as? CGFloat {
xf = CATransform3DScale(xf, scale, scale, 1.0)
hasValue = true
}
// LATER: Use "where"
if let scales = value["scale"] as? [CGFloat] {
if scales.count == 2 {
xf = CATransform3DScale(xf, scales[0], scales[1], 1.0)
}
hasValue = true
}
}
return hasValue ? xf : nil
}
return nil
}
static func parseSize(_ param:Any?, defaultValue:CGSize = CGSize(width: 0.0, height: 0.0), scale:CGSize) -> CGSize {
if let values = param as? [CGFloat], values.count == 2 {
return CGSize(width: values[0] * scale.width, height: values[1] * scale.height)
}
return CGSize(width: defaultValue.width * scale.width, height: defaultValue.height * scale.height)
}
static func parseFloat(_ param:Any?, defaultValue:Float = 1.0) -> Float {
if let value = param as? Float {
return value
}
return defaultValue
}
static func parseCGFloat(_ param:Any?, defaultValue:CGFloat = 0.0) -> CGFloat {
if let value = param as? CGFloat {
return value
}
return defaultValue
}
static func parseAndEvalBool(_ originator: SwipeNode, key: String, info: [String:Any]) -> Bool? {
var valObj: Any?
if let keyInfo = info[key] as? [String:Any], let valOfInfo = keyInfo["valueOf"] as? [String:Any] {
valObj = originator.getValue(originator, info:valOfInfo)
} else {
valObj = info[key]
}
if let val = valObj as? Bool {
return val
} else if let val = valObj as? Int {
return val != 0
} else {
return nil
}
}
//
// This function performs the "deep inheritance"
// Object property: Instance properties overrides Base properties
// Array property: Merge (inherit properties in case of "id" matches, otherwise, just append)
//
static func inheritProperties(_ object:[String:Any], baseObject:[String:Any]?) -> [String:Any] {
var ret = object
if let prototype = baseObject {
for (keyString, value) in prototype {
if ret[keyString] == nil {
// Only the baseObject has the property
ret[keyString] = value
} else if let arrayObject = ret[keyString] as? [[String:Any]], let arrayBase = value as? [[String:Any]] {
// Each has the property array. We need to merge them
var retArray = arrayBase
var idMap = [String:Int]()
for (index, item) in retArray.enumerated() {
if let key = item["id"] as? String {
idMap[key] = index
}
}
for item in arrayObject {
if let key = item["id"] as? String {
if let index = idMap[key] {
// id matches, merge them
retArray[index] = SwipeParser.inheritProperties(item, baseObject: retArray[index])
} else {
// no id match, just append
retArray.append(item)
}
} else {
// no id, just append
retArray.append(item)
}
}
ret[keyString] = retArray
} else if let objects = ret[keyString] as? [String:Any], let objectsBase = value as? [String:Any] {
// Each has the property objects. We need to merge them. Example: '"events" { }'
var retObjects = objectsBase
for (key, val) in objects {
retObjects[key] = val
}
ret[keyString] = retObjects
}
}
}
return ret
}
/*
static func imageWith(name:String) -> UIImage? {
var components = name.componentsSeparatedByString("/")
if components.count == 1 {
return UIImage(named: name)
}
let filename = components.last
components.removeLast()
let dir = components.joinWithSeparator("/")
if let path = NSBundle.mainBundle().pathForResource(filename, ofType: nil, inDirectory: dir) {
//NSLog("ScriptParset \(path)")
return UIImage(contentsOfFile: path)
}
return nil
}
static func imageSourceWith(name:String) -> CGImageSource? {
var components = name.componentsSeparatedByString("/")
let url:URL?
if components.count == 1 {
url = NSBundle.mainBundle().URLForResource(name, withExtension: nil)
} else {
let filename = components.last
components.removeLast()
let dir = components.joinWithSeparator("/")
url = NSBundle.mainBundle().URLForResource(filename!, withExtension: nil, subdirectory: dir)
}
if let urlImage = url {
return CGImageSourceCreateWithURL(urlImage, nil)
}
return nil
}
*/
static let regexPercent = try! NSRegularExpression(pattern: "^[0-9\\.]+%$", options: NSRegularExpression.Options.caseInsensitive)
static func parsePercent(_ value:String, full:CGFloat, defaultValue:CGFloat) -> CGFloat {
let num = regexPercent.numberOfMatches(in: value, options: NSRegularExpression.MatchingOptions(), range: NSMakeRange(0, value.characters.count))
if num == 1 {
return CGFloat((value as NSString).floatValue / 100.0) * full
}
return defaultValue
}
static func parsePercentAny(_ value:Any, full:CGFloat, defaultValue:CGFloat) -> CGFloat? {
if let f = value as? CGFloat {
return f
} else if let str = value as? String {
return SwipeParser.parsePercent(str, full: full, defaultValue: defaultValue)
}
return nil
}
static func parseFont(_ value:Any?, scale:CGSize, full:CGFloat) -> UIFont {
let fontSize = parseFontSize(value, full: full, defaultValue: 20, markdown: true)
let fontNames = parseFontName(value, markdown: true)
for name in fontNames {
if let font = UIFont(name: name, size: fontSize * scale.height) {
return font
}
}
return UIFont.systemFont(ofSize: fontSize * scale.height)
}
static func parseFontSize(_ value:Any?, full:CGFloat, defaultValue:CGFloat, markdown:Bool) -> CGFloat {
let key = markdown ? "size" : "fontSize"
if let info = value as? [String:Any] {
if let sizeValue = info[key] as? CGFloat {
return sizeValue
} else if let percent = info[key] as? String {
return SwipeParser.parsePercent(percent, full: full, defaultValue: defaultValue)
}
}
return defaultValue
}
static func parseFontName(_ value:Any?, markdown:Bool) -> [String] {
let key = markdown ? "name" : "fontName"
if let info = value as? [String:Any] {
if let name = info[key] as? String {
return [name]
} else if let names = info[key] as? [String] {
return names
}
}
return []
}
static func parseShadow(_ value:Any?, scale:CGSize) -> NSShadow {
let shadow = NSShadow()
if let info = value as? [String:Any] {
shadow.shadowOffset = SwipeParser.parseSize(info["offset"], defaultValue: CGSize(width: 1.0, height: 1.0), scale:scale)
shadow.shadowBlurRadius = SwipeParser.parseCGFloat(info["radius"], defaultValue: 2) * scale.width
shadow.shadowColor = UIColor(cgColor: SwipeParser.parseColor(info["color"], defaultColor: UIColor.black.cgColor))
}
return shadow
}
static func localizedString(_ params:[String:Any], langId:String?) -> String? {
if let key = langId,
let text = params[key] as? String {
return text
} else if let text = params["*"] as? String {
return text
}
return nil
}
static func preferredLangId(in languages: [[String : Any]]) -> String? {
// 1st, find a lang id matching system language.
// A lang id can be in "en-US", "en", "zh-Hans-CN" or "zh-Hans" formats (with/without a country code).
// "en-US" or "zh-Hans-CN" formats with a country code have higher priority to match than "en" or "zh-Hans" without a country code.
if let systemLanguage = NSLocale.preferredLanguages.first?.components(separatedBy: "-") {
for rangeEnd in systemLanguage.indices.reversed() {
let langId = systemLanguage[0...rangeEnd].joined(separator: "-")
if languages.contains(where: { $0["id"] as? String == langId }) {
return langId
}
}
}
// 2nd, use the default lang id ("*") if no lang id matches, and the default id exists.
if languages.contains(where: { $0["id"] as? String == "*" }) {
return "*"
}
// At last, use the first lang id in the language list if still no lang id is specified.
if let langId = languages.first?["id"] as? String {
return langId
}
return nil
}
}
| mit | eb0d3a95780892f1f9c0b365bdcaafce | 40.092965 | 163 | 0.512687 | 4.514215 | false | false | false | false |
ngageoint/mage-ios | Mage/DropdownFieldView.swift | 1 | 4361 | //
// DropdownFieldView.swift
// MAGE
//
// Created by Daniel Barela on 5/27/20.
// Copyright © 2020 National Geospatial Intelligence Agency. All rights reserved.
//
import Foundation
import MaterialComponents.MDCTextField;
class DropdownFieldView : BaseFieldView {
lazy var textField: MDCFilledTextField = {
let textField = MDCFilledTextField(frame: CGRect(x: 0, y: 0, width: 200, height: 100));
textField.trailingView = UIImageView(image: UIImage(named: "arrow_drop_down"));
textField.accessibilityLabel = "\(field[FieldKey.name.key] as? String ?? "") value"
textField.trailingViewMode = .always;
textField.leadingAssistiveLabel.text = " ";
if (value != nil) {
textField.text = getDisplayValue();
}
textField.sizeToFit();
return textField;
}()
override func applyTheme(withScheme scheme: MDCContainerScheming?) {
guard let scheme = scheme else {
return
}
super.applyTheme(withScheme: scheme);
textField.applyTheme(withScheme: scheme);
textField.trailingView?.tintColor = scheme.colorScheme.onSurfaceColor.withAlphaComponent(0.6);
}
required init(coder aDecoder: NSCoder) {
fatalError("This class does not support NSCoding")
}
convenience init(field: [String: Any], editMode: Bool = true, delegate: (ObservationFormFieldListener & FieldSelectionDelegate)? = nil) {
self.init(field: field, editMode: editMode, delegate: delegate, value: nil);
}
init(field: [String: Any], editMode: Bool = true, delegate: (ObservationFormFieldListener & FieldSelectionDelegate)? = nil, value: String?) {
super.init(field: field, delegate: delegate, value: value, editMode: editMode);
self.addFieldView();
}
func addFieldView() {
if (self.editMode) {
viewStack.addArrangedSubview(textField);
let tapView = addTapRecognizer();
tapView.accessibilityLabel = field[FieldKey.name.key] as? String;
setPlaceholder(textField: textField);
} else {
viewStack.addArrangedSubview(fieldNameLabel);
viewStack.addArrangedSubview(fieldValue);
fieldValue.text = getDisplayValue();
}
}
func getDisplayValue() -> String? {
return getValue();
}
override func setValue(_ value: Any?) {
self.value = value
self.editMode ? (textField.text = getDisplayValue()) : (fieldValue.text = getDisplayValue());
}
func getValue() -> String? {
return value as? String;
}
override func isEmpty() -> Bool {
return (textField.text ?? "").count == 0;
}
override func setValid(_ valid: Bool) {
super.setValid(valid);
if (valid) {
textField.leadingAssistiveLabel.text = " ";
if let scheme = scheme {
textField.applyTheme(withScheme: scheme);
}
} else {
textField.applyErrorTheme(withScheme: globalErrorContainerScheme());
textField.leadingAssistiveLabel.text = getErrorMessage();
}
}
func getInvalidChoice() -> String? {
var choiceStrings: [String] = []
if let choices = self.field[FieldKey.choices.key] as? [[String: Any]] {
for choice in choices {
if let choiceString = choice[FieldKey.title.key] as? String {
choiceStrings.append(choiceString)
}
}
}
if let value = self.value as? String {
if (!choiceStrings.contains(value)) {
return value;
}
}
return nil;
}
override func isValid(enforceRequired: Bool = false) -> Bool {
if getInvalidChoice() != nil {
return false;
}
// verify the choices are in the list of choices in case defaults were set
return super.isValid(enforceRequired: enforceRequired);
}
override func getErrorMessage() -> String {
if let invalidChoice = getInvalidChoice() {
return "\(invalidChoice) is not a valid option."
}
return ((field[FieldKey.title.key] as? String) ?? "Field ") + " is required";
}
}
| apache-2.0 | 2b9e5e6058caaef7014e20cc7544b758 | 33.0625 | 145 | 0.596101 | 4.849833 | false | false | false | false |
nanthi1990/MaterialKit | Source/TextView.swift | 3 | 4473 | //
// Copyright (C) 2015 GraphKit, Inc. <http://graphkit.io> and other GraphKit contributors.
//
// This program is free software: you can redistribute it and/or modify
// it under the terms of the GNU Affero General Public License as published
// by the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// This program is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU Affero General Public License for more details.
//
// You should have received a copy of the GNU Affero General Public License
// along with this program located at the root of the software package
// in a file called LICENSE. If not, see <http://www.gnu.org/licenses/>.
//
import UIKit
public class TextView: UITextView {
//
// :name: layoutConstraints
//
internal lazy var layoutConstraints: Array<NSLayoutConstraint> = Array<NSLayoutConstraint>()
/**
:name: init
*/
public required init(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)
prepareView()
}
/**
:name: init
*/
public override init(frame: CGRect, textContainer: NSTextContainer?) {
super.init(frame: frame, textContainer: textContainer)
if CGRectZero == frame {
setTranslatesAutoresizingMaskIntoConstraints(false)
}
prepareView()
}
//
// :name: deinit
// :description: Notification observer removed from UITextView.
//
deinit {
NSNotificationCenter.defaultCenter().removeObserver(self, name: UITextViewTextDidChangeNotification, object: nil)
}
/**
:name: placeholder
:description: The placeholder label string.
*/
public var placeholderLabel: UILabel? {
didSet {
if let p = placeholderLabel {
p.setTranslatesAutoresizingMaskIntoConstraints(false)
p.font = font
p.textAlignment = textAlignment
p.numberOfLines = 0
p.backgroundColor = MaterialTheme.clear.color
addSubview(p)
updateLabelConstraints()
textViewTextDidChange()
}
}
}
/**
:name: text
:description: When set, updates the placeholder text.
*/
public override var text: String! {
didSet {
textViewTextDidChange()
}
}
/**
:name: attributedText
:description: When set, updates the placeholder attributedText.
*/
public override var attributedText: NSAttributedString! {
didSet {
textViewTextDidChange()
}
}
/**
:name: textContainerInset
:description: When set, updates the placeholder constraints.
*/
public override var textContainerInset: UIEdgeInsets {
didSet {
updateLabelConstraints()
}
}
public override func layoutSubviews() {
super.layoutSubviews()
placeholderLabel?.preferredMaxLayoutWidth = textContainer.size.width - textContainer.lineFragmentPadding * 2
}
//
// :name: textViewTextDidChange
// :description: Updates the label visibility when text is empty or not.
//
internal func textViewTextDidChange() {
if let p = placeholderLabel {
p.hidden = !text.isEmpty
}
}
//
// :name: prepareView
// :description: Sets up the common initilized values.
//
private func prepareView() {
// label needs to be added to the view
// hierarchy before setting insets
textContainerInset = UIEdgeInsetsMake(16, 16, 16, 16)
backgroundColor = MaterialTheme.clear.color
NSNotificationCenter.defaultCenter().addObserver(self, selector: "textViewTextDidChange", name: UITextViewTextDidChangeNotification, object: nil)
updateLabelConstraints()
}
//
// :name: updateLabelConstraints
// :description: Updates the placeholder constraints.
//
private func updateLabelConstraints() {
if let p = placeholderLabel {
NSLayoutConstraint.deactivateConstraints(layoutConstraints)
layoutConstraints = Layout.constraint("H:|-(left)-[placeholderLabel]-(right)-|",
options: nil,
metrics: [
"left": textContainerInset.left + textContainer.lineFragmentPadding,
"right": textContainerInset.right + textContainer.lineFragmentPadding
], views: [
"placeholderLabel": p
])
layoutConstraints += Layout.constraint("V:|-(top)-[placeholderLabel]-(>=bottom)-|",
options: nil,
metrics: [
"top": textContainerInset.top,
"bottom": textContainerInset.bottom
],
views: [
"placeholderLabel": p
])
NSLayoutConstraint.activateConstraints(layoutConstraints)
}
}
}
| agpl-3.0 | 99322e9d084b19a88d18558863bee5bf | 26.95625 | 147 | 0.707802 | 4.184284 | false | false | false | false |
nickqiao/NKBill | NKBill/Controller/NKTabBarController.swift | 1 | 1018 | //
// NKBaseTabBarController.swift
// NKBill
//
// Created by nick on 16/1/30.
// Copyright © 2016年 NickChen. All rights reserved.
//
import UIKit
class NKTabBarController: UITabBarController {
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
self.delegate = self
self.tabBar.items![0].selectedImage = UIImage(named: "index")
}
override func tabBar(tabBar: UITabBar, didSelectItem item: UITabBarItem) {
if item.title == "记一笔" {
performSegueWithIdentifier("compose", sender: nil)
}
}
}
extension NKTabBarController: UITabBarControllerDelegate {
func tabBarController(tabBarController: UITabBarController, shouldSelectViewController viewController: UIViewController) -> Bool {
if viewController == tabBarController.viewControllers![2] {
return false
}else {
return true
}
}
}
| apache-2.0 | 62a501ce610bd479221485c0f97b964a | 22.465116 | 134 | 0.635282 | 4.99505 | false | false | false | false |
AirChen/ACSwiftDemoes | Target12-TableViewAnimate/SecondTableViewController.swift | 1 | 2765 | //
// SecondTableViewController.swift
// ACSwiftTest
//
// Created by Air_chen on 2016/11/6.
// Copyright © 2016年 Air_chen. All rights reserved.
//
import UIKit
class SecondTableViewController: UITableViewController {
var tableData = ["Read 3 article on Medium", "Cleanup bedroom", "Go for a run", "Hit the gym", "Build another swift project", "Movement training", "Fix the layout problem of a client project", "Write the experience of #30daysSwift", "Inbox Zero", "Booking the ticket to Chengdu", "Test the Adobe Project Comet", "Hop on a call to mom"]
override func viewDidLoad() {
super.viewDidLoad()
self.tableView.separatorStyle = UITableViewCellSeparatorStyle.none
}
override func viewWillAppear(_ animated: Bool) {
super.viewWillAppear(animated)
self.loadCells()
}
func loadCells() {
self.tableView.reloadData()
let cells = self.tableView.visibleCells
for (index,acell) in cells.enumerated() {
let cell = acell as! ACTableViewCell
cell.transform = CGAffineTransform.init(translationX: 0, y: self.tableView.bounds.height)
UIView.animate(withDuration: 0.08*Double(index), animations: {
cell.transform = CGAffineTransform.identity
})
}
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
// MARK: - Table view data source
override func numberOfSections(in tableView: UITableView) -> Int {
// #warning Incomplete implementation, return the number of sections
return 1
}
override func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
// #warning Incomplete implementation, return the number of rows
return self.tableData.count
}
override func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "Cell")
cell?.textLabel?.backgroundColor = UIColor.clear
cell?.textLabel?.text = self.tableData[indexPath.row]
return cell!
}
func colorforIndex(index: Int) -> UIColor {
let itemCount = tableData.count - 1
let color = (CGFloat(index) / CGFloat(itemCount)) * 0.6
return UIColor(red: 1.0, green: color, blue: 0.0, alpha: 1.0)
}
override func tableView(_ tableView: UITableView, willDisplay cell: UITableViewCell, forRowAt indexPath: IndexPath) {
cell.backgroundColor = self.colorforIndex(index: indexPath.row)
}
}
| mit | 3a820f642ea9ac60981cb9dfcb5760ab | 33.525 | 339 | 0.646271 | 4.914591 | false | false | false | false |
soapyigu/LeetCode_Swift | LinkedList/MergeTwoSortedLists.swift | 1 | 1019 | /**
* Question Link: https://leetcode.com/problems/merge-two-sorted-lists/
* Primary idea: Dummy Node to traverse two lists, compare two nodes and point to the right one
* Time Complexity: O(n), Space Complexity: O(1)
*
* Definition for singly-linked list.
* public class ListNode {
* public var val: Int
* public var next: ListNode?
* public init(_ val: Int) {
* self.val = val
* self.next = nil
* }
* }
*/
class MergeTwoSortedLists {
func mergeTwoLists(l1: ListNode?, _ l2: ListNode?) -> ListNode? {
let dummy = ListNode(0)
var node = dummy
var l1 = l1
var l2 = l2
while l1 != nil && l2 != nil {
if l1!.val < l2!.val {
node.next = l1
l1 = l1!.next
} else {
node.next = l2
l2 = l2!.next
}
node = node.next!
}
node.next = l1 ?? l2
return dummy.next
}
} | mit | 904d7d9d762938c107149a5745fb118d | 24.5 | 95 | 0.491658 | 3.732601 | false | false | false | false |
warnerbros/cpe-manifest-ios-data | Source/AppDataSet.swift | 1 | 3940 | //
// AppDataSet.swift
//
import Foundation
import SWXMLHash
open class AppDataSet {
private struct Attributes {
static let Name = "Name"
static let AppDataType = "type"
}
private struct Elements {
static let ManifestAppData = "ManifestAppData"
static let NVPair = "NVPair"
static let Text = "Text"
}
private enum AppDataType: String {
case product = "PRODUCT"
case person = "PERSON"
case location = "LOCATION"
}
// Inventory
public var locations: [String: AppDataItemLocation]?
public var products: [String: AppDataItemProduct]?
public var people: [String: AppDataItem]?
public var imageCache: [String: UIImage]?
init(indexer: XMLIndexer) throws {
// ManifestAppData
guard indexer.hasElement(Elements.ManifestAppData) else {
throw ManifestError.missingRequiredChildElement(name: Elements.ManifestAppData, element: indexer.element)
}
for indexer in indexer[Elements.ManifestAppData].all {
if try indexer[Elements.NVPair].withAttribute(Attributes.Name, Attributes.AppDataType)[Elements.Text].value() == AppDataType.product.rawValue {
if products == nil {
products = [String: AppDataItemProduct]()
}
let product = try AppDataItemProduct(indexer: indexer)
products![product.id] = product
} else if try indexer[Elements.NVPair].withAttribute(Attributes.Name, Attributes.AppDataType)[Elements.Text].value() == AppDataType.person.rawValue {
if people == nil {
people = [String: AppDataItem]()
}
let person = try AppDataItem(indexer: indexer)
people![person.id] = person
} else {
if locations == nil {
locations = [String: AppDataItemLocation]()
}
let location = try AppDataItemLocation(indexer: indexer)
locations![location.id] = location
}
}
}
open func postProcess() {
if let locations = locations {
for location in Array(locations.values) {
if let imageID = location.iconImageID, let url = CPEXMLSuite.current?.manifest.imageWithID(imageID)?.url {
_ = UIImageRemoteLoader.loadImage(url, completion: { [weak self] (image) in
if let strongSelf = self {
if strongSelf.imageCache == nil {
strongSelf.imageCache = [String: UIImage]()
}
strongSelf.imageCache![imageID] = image
}
})
}
}
}
if let appDataPeople = people {
if let people = CPEXMLSuite.current?.manifest.people {
for person in people {
if let appDataID = person.appDataID, let appDataItem = appDataPeople[appDataID] {
person.biographyHeader = appDataItem.descriptionHeader
person.biography = appDataItem.description
if let pictures = appDataItem.pictures {
person.pictureGroup = PictureGroup(pictures: pictures)
}
person.detailsLoaded = true
}
}
}
self.people = nil
}
}
open func cachedImageWithID(_ id: String?) -> UIImage? {
return (id != nil ? imageCache?[id!] : nil)
}
open func locationWithID(_ id: String?) -> AppDataItemLocation? {
return (id != nil ? locations?[id!] : nil)
}
open func productWithID(_ id: String?) -> AppDataItemProduct? {
return (id != nil ? products?[id!] : nil)
}
}
| apache-2.0 | e92cbd1908784dabb106494088008a55 | 33.867257 | 161 | 0.544924 | 5.260347 | false | false | false | false |
iOSTestApps/WordPress-iOS | WordPress/WordPressTodayWidget/TodayViewController.swift | 10 | 5003 | import UIKit
import NotificationCenter
class TodayViewController: UIViewController, NCWidgetProviding {
@IBOutlet var siteNameLabel: UILabel!
@IBOutlet var visitorsCountLabel: UILabel!
@IBOutlet var visitorsLabel: UILabel!
@IBOutlet var viewsCountLabel: UILabel!
@IBOutlet var viewsLabel: UILabel!
var siteName: String = ""
var visitorCount: String = ""
var viewCount: String = ""
var siteId: NSNumber?
override func viewDidLoad() {
super.viewDidLoad()
let sharedDefaults = NSUserDefaults(suiteName: WPAppGroupName)!
self.siteId = sharedDefaults.objectForKey(WPStatsTodayWidgetUserDefaultsSiteIdKey) as! NSNumber?
visitorsLabel?.text = NSLocalizedString("Visitors", comment: "Stats Visitors Label")
viewsLabel?.text = NSLocalizedString("Views", comment: "Stats Views Label")
}
override func viewWillDisappear(animated: Bool) {
super.viewWillDisappear(animated)
// Manual state restoration
var userDefaults = NSUserDefaults.standardUserDefaults()
userDefaults.setObject(self.siteName, forKey: WPStatsTodayWidgetUserDefaultsSiteNameKey)
userDefaults.setObject(self.visitorCount, forKey: WPStatsTodayWidgetUserDefaultsVisitorCountKey)
userDefaults.setObject(self.viewCount, forKey: WPStatsTodayWidgetUserDefaultsViewCountKey)
}
override func viewWillAppear(animated: Bool) {
super.viewWillAppear(animated)
// Manual state restoration
let sharedDefaults = NSUserDefaults(suiteName: WPAppGroupName)!
self.siteName = sharedDefaults.stringForKey(WPStatsTodayWidgetUserDefaultsSiteNameKey) ?? ""
let userDefaults = NSUserDefaults.standardUserDefaults()
self.visitorCount = userDefaults.stringForKey(WPStatsTodayWidgetUserDefaultsVisitorCountKey) ?? "0"
self.viewCount = userDefaults.stringForKey(WPStatsTodayWidgetUserDefaultsViewCountKey) ?? "0"
self.siteNameLabel?.text = self.siteName
self.visitorsCountLabel?.text = self.visitorCount
self.viewsCountLabel?.text = self.viewCount
}
@IBAction func launchContainingApp() {
self.extensionContext!.openURL(NSURL(string: "\(WPCOM_SCHEME)://viewstats?siteId=\(siteId!)")!, completionHandler: nil)
}
func widgetPerformUpdateWithCompletionHandler(completionHandler: ((NCUpdateResult) -> Void)!) {
// Perform any setup necessary in order to update the view.
// If an error is encoutered, use NCUpdateResult.Failed
// If there's no update required, use NCUpdateResult.NoData
// If there's an update, use NCUpdateResult.NewData
let sharedDefaults = NSUserDefaults(suiteName: WPAppGroupName)!
let siteId = sharedDefaults.objectForKey(WPStatsTodayWidgetUserDefaultsSiteIdKey) as! NSNumber?
self.siteName = sharedDefaults.stringForKey(WPStatsTodayWidgetUserDefaultsSiteNameKey) ?? ""
let timeZoneName = sharedDefaults.stringForKey(WPStatsTodayWidgetUserDefaultsSiteTimeZoneKey)
let oauth2Token = self.getOAuth2Token()
if siteId == nil || timeZoneName == nil || oauth2Token == nil {
WPDDLogWrapper.logError("Missing site ID, timeZone or oauth2Token")
let bundle = NSBundle(forClass: TodayViewController.classForCoder())
NCWidgetController.widgetController().setHasContent(false, forWidgetWithBundleIdentifier: bundle.bundleIdentifier)
completionHandler(NCUpdateResult.Failed)
return
}
let timeZone = NSTimeZone(name: timeZoneName!)
var statsService: WPStatsService = WPStatsService(siteId: siteId, siteTimeZone: timeZone, oauth2Token: oauth2Token, andCacheExpirationInterval:0)
statsService.retrieveTodayStatsWithCompletionHandler({ (wpStatsSummary: StatsSummary!, error: NSError!) -> Void in
WPDDLogWrapper.logInfo("Downloaded data in the Today widget")
self.visitorCount = wpStatsSummary.visitors
self.viewCount = wpStatsSummary.views
self.siteNameLabel?.text = self.siteName
self.visitorsCountLabel?.text = self.visitorCount
self.viewsCountLabel?.text = self.viewCount
completionHandler(NCUpdateResult.NewData)
}, failureHandler: { (error) -> Void in
WPDDLogWrapper.logError("\(error)")
completionHandler(NCUpdateResult.Failed)
})
}
func getOAuth2Token() -> String? {
var error:NSError?
var oauth2Token:NSString? = SFHFKeychainUtils.getPasswordForUsername(WPStatsTodayWidgetOAuth2TokenKeychainUsername, andServiceName: WPStatsTodayWidgetOAuth2TokenKeychainServiceName, accessGroup: WPStatsTodayWidgetOAuth2TokenKeychainAccessGroup, error: &error)
return oauth2Token as String?
}
} | gpl-2.0 | 563e128c5b33f0ea084c20b2154b71c3 | 45.766355 | 267 | 0.697981 | 5.659502 | false | false | false | false |
alexktchen/rescue.iOS | Rescue/AppDelegate.swift | 1 | 7463 | //
// AppDelegate.swift
// Rescue
//
// Created by Alex Chen on 2015/1/29.
// Copyright (c) 2015年 KKAwesome. All rights reserved.
//
import UIKit
import CoreData
import CoreLocation
@UIApplicationMain
class AppDelegate: UIResponder, UIApplicationDelegate {
var window: UIWindow?
var locationManager: CLLocationManager?
let reachability = Reachability.reachabilityForInternetConnection()
func application(application: UIApplication, didFinishLaunchingWithOptions launchOptions: [NSObject: AnyObject]?) -> Bool {
// let service: StorageService = StorageService()
locationManager = CLLocationManager()
locationManager?.requestWhenInUseAuthorization()
let defaults = NSUserDefaults.standardUserDefaults()
if let name = defaults.stringForKey("userName")
{
println(name)
}
else{
defaults.setObject(UIDevice.currentDevice().name, forKey: "userName")
}
// regest the reachbility class noitfy to the AppDelegate
NSNotificationCenter.defaultCenter().addObserver(self, selector: "reachabilityChanged:", name: ReachabilityChangedNotification, object: reachability)
reachability.startNotifier()
return true
}
/*
occure when the 3G/4G is not aviablab or wifi can't connect to internet
*/
func reachabilityChanged(note: NSNotification) {
let reachability = note.object as Reachability
if reachability.isReachable() {
if reachability.isReachableViaWiFi() {
println("Reachable via WiFi")
} else {
println("Reachable via Cellular")
}
}
else {
println("Not reachable")
}
}
func applicationWillResignActive(application: UIApplication) {
// Sent when the application is about to move from active to inactive state. This can occur for certain types of temporary interruptions (such as an incoming phone call or SMS message) or when the user quits the application and it begins the transition to the background state.
// Use this method to pause ongoing tasks, disable timers, and throttle down OpenGL ES frame rates. Games should use this method to pause the game.
}
func applicationDidEnterBackground(application: UIApplication) {
// Use this method to release shared resources, save user data, invalidate timers, and store enough application state information to restore your application to its current state in case it is terminated later.
// If your application supports background execution, this method is called instead of applicationWillTerminate: when the user quits.
}
func applicationWillEnterForeground(application: UIApplication) {
// Called as part of the transition from the background to the inactive state; here you can undo many of the changes made on entering the background.
}
func applicationDidBecomeActive(application: UIApplication) {
// Restart any tasks that were paused (or not yet started) while the application was inactive. If the application was previously in the background, optionally refresh the user interface.
}
func applicationWillTerminate(application: UIApplication) {
// Called when the application is about to terminate. Save data if appropriate. See also applicationDidEnterBackground:.
}
// MARK: - Core Data stack
lazy var applicationDocumentsDirectory: NSURL = {
// The directory the application uses to store the Core Data store file. This code uses a directory named "KKAwesome.test" in the application's documents Application Support directory.
let urls = NSFileManager.defaultManager().URLsForDirectory(.DocumentDirectory, inDomains: .UserDomainMask)
return urls[urls.count-1] as NSURL
}()
lazy var managedObjectModel: NSManagedObjectModel = {
// The managed object model for the application. This property is not optional. It is a fatal error for the application not to be able to find and load its model.
let modelURL = NSBundle.mainBundle().URLForResource("Message", withExtension: "momd")!
return NSManagedObjectModel(contentsOfURL: modelURL)!
}()
lazy var persistentStoreCoordinator: NSPersistentStoreCoordinator? = {
// The persistent store coordinator for the application. This implementation creates and return a coordinator, having added the store for the application to it. This property is optional since there are legitimate error conditions that could cause the creation of the store to fail.
// Create the coordinator and store
var coordinator: NSPersistentStoreCoordinator? = NSPersistentStoreCoordinator(managedObjectModel: self.managedObjectModel)
let url = self.applicationDocumentsDirectory.URLByAppendingPathComponent("test.sqlite")
var error: NSError? = nil
var failureReason = "There was an error creating or loading the application's saved data."
if coordinator!.addPersistentStoreWithType(NSSQLiteStoreType, configuration: nil, URL: url, options: nil, error: &error) == nil {
coordinator = nil
// Report any error we got.
let dict = NSMutableDictionary()
dict[NSLocalizedDescriptionKey] = "Failed to initialize the application's saved data"
dict[NSLocalizedFailureReasonErrorKey] = failureReason
dict[NSUnderlyingErrorKey] = error
error = NSError(domain: "YOUR_ERROR_DOMAIN", code: 9999, userInfo: dict)
// Replace this with code to handle the error appropriately.
// abort() causes the application to generate a crash log and terminate. You should not use this function in a shipping application, although it may be useful during development.
NSLog("Unresolved error \(error), \(error!.userInfo)")
abort()
}
return coordinator
}()
lazy var managedObjectContext: NSManagedObjectContext? = {
// Returns the managed object context for the application (which is already bound to the persistent store coordinator for the application.) This property is optional since there are legitimate error conditions that could cause the creation of the context to fail.
let coordinator = self.persistentStoreCoordinator
if coordinator == nil {
return nil
}
var managedObjectContext = NSManagedObjectContext()
managedObjectContext.persistentStoreCoordinator = coordinator
return managedObjectContext
}()
// MARK: - Core Data Saving support
func saveContext () {
if let moc = self.managedObjectContext {
var error: NSError? = nil
if moc.hasChanges && !moc.save(&error) {
// Replace this implementation with code to handle the error appropriately.
// abort() causes the application to generate a crash log and terminate. You should not use this function in a shipping application, although it may be useful during development.
NSLog("Unresolved error \(error), \(error!.userInfo)")
abort()
}
}
}
}
| mit | 118a1a3c5b0cfc4b2ba1713d4965e855 | 46.221519 | 290 | 0.680472 | 5.98316 | false | false | false | false |
KrisYu/Octobook | Pods/Fuzi/Sources/Node.swift | 5 | 6146 | // Node.swift
// Copyright (c) 2015 Ce Zheng
//
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in
// all copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
// THE SOFTWARE.
import Foundation
import libxml2
/// Define a Swifty typealias for libxml's node type enum
public typealias XMLNodeType = xmlElementType
// MARK: - Give a Swifty name to each enum case of XMLNodeType
extension XMLNodeType {
/// Element
public static var Element: xmlElementType { return XML_ELEMENT_NODE }
/// Attribute
public static var Attribute: xmlElementType { return XML_ATTRIBUTE_NODE }
/// Text
public static var Text: xmlElementType { return XML_TEXT_NODE }
/// CData Section
public static var CDataSection: xmlElementType { return XML_CDATA_SECTION_NODE }
/// Entity Reference
public static var EntityRef: xmlElementType { return XML_ENTITY_REF_NODE }
/// Entity
public static var Entity: xmlElementType { return XML_ENTITY_NODE }
/// Pi
public static var Pi: xmlElementType { return XML_PI_NODE }
/// Comment
public static var Comment: xmlElementType { return XML_COMMENT_NODE }
/// Document
public static var Document: xmlElementType { return XML_DOCUMENT_NODE }
/// Document Type
public static var DocumentType: xmlElementType { return XML_DOCUMENT_TYPE_NODE }
/// Document Fragment
public static var DocumentFrag: xmlElementType { return XML_DOCUMENT_FRAG_NODE }
/// Notation
public static var Notation: xmlElementType { return XML_NOTATION_NODE }
/// HTML Document
public static var HtmlDocument: xmlElementType { return XML_HTML_DOCUMENT_NODE }
/// DTD
public static var DTD: xmlElementType { return XML_DTD_NODE }
/// Element Declaration
public static var ElementDecl: xmlElementType { return XML_ELEMENT_DECL }
/// Attribute Declaration
public static var AttributeDecl: xmlElementType { return XML_ATTRIBUTE_DECL }
/// Entity Declaration
public static var EntityDecl: xmlElementType { return XML_ENTITY_DECL }
/// Namespace Declaration
public static var NamespaceDecl: xmlElementType { return XML_NAMESPACE_DECL }
/// XInclude Start
public static var XIncludeStart: xmlElementType { return XML_XINCLUDE_START }
/// XInclude End
public static var XIncludeEnd: xmlElementType { return XML_XINCLUDE_END }
/// DocbDocument
public static var DocbDocument: xmlElementType { return XML_DOCB_DOCUMENT_NODE }
}
infix operator ~=
/**
For supporting pattern matching of those enum case alias getters for XMLNodeType
- parameter lhs: left hand side
- parameter rhs: right hand side
- returns: true if both sides equals
*/
public func ~=(lhs: XMLNodeType, rhs: XMLNodeType) -> Bool {
return lhs.rawValue == rhs.rawValue
}
/// Base class for all XML nodes
open class XMLNode {
/// The document containing the element.
open unowned let document: XMLDocument
/// The type of the XMLNode
open var type: XMLNodeType {
return cNode.pointee.type
}
/// The element's line number.
open fileprivate(set) lazy var lineNumber: Int = {
return xmlGetLineNo(self.cNode)
}()
// MARK: - Accessing Parent and Sibling Elements
/// The element's parent element.
open fileprivate(set) lazy var parent: XMLElement? = {
return XMLElement(cNode: self.cNode.pointee.parent, document: self.document)
}()
/// The element's next sibling.
open fileprivate(set) lazy var previousSibling: XMLElement? = {
return XMLElement(cNode: self.cNode.pointee.prev, document: self.document)
}()
/// The element's previous sibling.
open fileprivate(set) lazy var nextSibling: XMLElement? = {
return XMLElement(cNode: self.cNode.pointee.next, document: self.document)
}()
// MARK: - Accessing Contents
/// Whether this is a HTML node
open var isHTML: Bool {
return UInt32(self.cNode.pointee.doc.pointee.properties) & XML_DOC_HTML.rawValue == XML_DOC_HTML.rawValue
}
/// A string representation of the element's value.
open fileprivate(set) lazy var stringValue : String = {
let key = xmlNodeGetContent(self.cNode)
let stringValue = ^-^key ?? ""
xmlFree(key)
return stringValue
}()
/// The raw XML string of the element.
open fileprivate(set) lazy var rawXML: String = {
let buffer = xmlBufferCreate()
if self.isHTML {
htmlNodeDump(buffer, self.cNode.pointee.doc, self.cNode)
} else {
xmlNodeDump(buffer, self.cNode.pointee.doc, self.cNode, 0, 0)
}
let dumped = ^-^xmlBufferContent(buffer) ?? ""
xmlBufferFree(buffer)
return dumped
}()
/// Convert this node to XMLElement if it is an element node
open func toElement() -> XMLElement? {
return self as? XMLElement
}
internal let cNode: xmlNodePtr
internal init(cNode: xmlNodePtr, document: XMLDocument) {
self.cNode = cNode
self.document = document
}
}
extension XMLNode: Equatable {}
/**
Determine whether two nodes are the same
- parameter lhs: XMLNode on the left
- parameter rhs: XMLNode on the right
- returns: whether lhs and rhs are equal
*/
public func ==(lhs: XMLNode, rhs: XMLNode) -> Bool {
return lhs.cNode == rhs.cNode
}
| mit | 48f24ed942d1142229168d99b7dc1f93 | 34.94152 | 109 | 0.714123 | 4.355776 | false | false | false | false |
bazelbuild/tulsi | src/Tulsi/UIMessage.swift | 1 | 1778 | // Copyright 2016 The Tulsi Authors. All rights reserved.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
import Cocoa
import TulsiGenerator
/// Defines an object that holds an array of UIMessages.
protocol MessageLogProtocol: AnyObject {
var messages: [UIMessage] { get }
}
/// Models a single user-facing message.
final class UIMessage: NSObject, NSPasteboardWriting {
@objc dynamic let text: String
@objc dynamic let messagePriority: TulsiGenerator.LogMessagePriority
let timestamp = Date()
init(text: String, type: TulsiGenerator.LogMessagePriority) {
self.text = text
self.messagePriority = type
}
// MARK: - NSPasteboardWriting
func writableTypes(for pasteboard: NSPasteboard) -> [NSPasteboard.PasteboardType] {
return [NSPasteboard.PasteboardType.string]
}
func pasteboardPropertyList(forType type: NSPasteboard.PasteboardType) -> Any? {
if type == NSPasteboard.PasteboardType.string {
let timeString = DateFormatter.localizedString(from: timestamp,
dateStyle: .none,
timeStyle: .medium)
return "[\(timeString)](\(messagePriority.rawValue)): \(text)"
}
return nil
}
}
| apache-2.0 | 33fbc52315d5eeae618d3dd86e1efce4 | 34.56 | 85 | 0.687289 | 4.792453 | false | false | false | false |
ascode/pmn | AnimationDemo/Controller/ClockUseEasingFuncViewController.swift | 1 | 6001 | //
// ClockUseEasingFuncViewController.swift
// AnimationDemo
//
// Created by 金飞 on 2016/10/18.
// Copyright © 2016年 JL. All rights reserved.
//
import UIKit
class ClockUseEasingFuncViewController: UIViewController {
var secLayer: CALayer!
var timer: Timer!
static var i: Double = Double(0)
override func viewDidLoad() {
super.viewDidLoad()
let clockPanel: UIView = UIView()
clockPanel.frame = CGRect(x: 0, y: 0, width: 300, height: 300)
clockPanel.center = self.view.center
clockPanel.layer.borderWidth = 1
clockPanel.layer.borderColor = UIColor.white.cgColor
clockPanel.layer.cornerRadius = 150
clockPanel.layer.masksToBounds = true
self.view.addSubview(clockPanel)
//设置指针
secLayer = CALayer()
secLayer.anchorPoint = CGPoint(x: 0, y: 0)
secLayer.frame = CGRect(x: 150, y: 150, width: 1, height: 130)
secLayer.backgroundColor = UIColor.white.cgColor
clockPanel.layer.addSublayer(secLayer)
/*1、参数repeats是指定是否循环执行,YES将循环,NO将只执行一次。
2、timerWithTimeInterval这两个类方法创建出来的对象如果不用 addTimer: forMode方法手动加入主循环池中,将不会循环执行。并且如果不手动调用fair,则定时器不会启动。
3、scheduledTimerWithTimeInterval这两个方法不需要手动调用fair,会自动执行,并且自动加入主循环池。
4、init方法需要手动加入循环池,它会在设定的启动时间启动。*/
timer = Timer(timeInterval: 1, target: self, selector: #selector(ClockUseEasingFuncViewController.timerEvent), userInfo: nil, repeats: true)
RunLoop.main.add(timer, forMode: RunLoopMode.defaultRunLoopMode)
timer.fire()
let btnStart: UIButton = UIButton()
btnStart.frame = CGRect(x: UIScreen.main.bounds.width * 0/3, y: UIScreen.main.bounds.height - 50, width: UIScreen.main.bounds.width / 3, height: 50)
btnStart.setTitle("播放", for: UIControlState.normal)
btnStart.layer.borderColor = UIColor.white.cgColor
btnStart.layer.borderWidth = 3
btnStart.layer.cornerRadius = 3
btnStart.layer.masksToBounds = true
btnStart.backgroundColor = UIColor.blue
btnStart.addTarget(self, action: #selector(ImageViewAnimationViewController.btnStartPressed), for: UIControlEvents.touchUpInside)
self.view.addSubview(btnStart)
let btnStop: UIButton = UIButton()
btnStop.frame = CGRect(x: UIScreen.main.bounds.width * 1/3, y: UIScreen.main.bounds.height - 50, width: UIScreen.main.bounds.width / 3, height: 50)
btnStop.setTitle("停止", for: UIControlState.normal)
btnStop.layer.borderColor = UIColor.white.cgColor
btnStop.layer.borderWidth = 3
btnStop.layer.cornerRadius = 3
btnStop.layer.masksToBounds = true
btnStop.backgroundColor = UIColor.blue
btnStop.addTarget(self, action: #selector(ImageViewAnimationViewController.btnStopPressed), for: UIControlEvents.touchUpInside)
self.view.addSubview(btnStop)
let btnBack: UIButton = UIButton()
btnBack.frame = CGRect(x: UIScreen.main.bounds.width * 2/3, y: UIScreen.main.bounds.height - 50, width: UIScreen.main.bounds.width / 3, height: 50)
btnBack.setTitle("返回", for: UIControlState.normal)
btnBack.layer.borderColor = UIColor.white.cgColor
btnBack.layer.borderWidth = 3
btnBack.layer.cornerRadius = 3
btnBack.layer.masksToBounds = true
btnBack.backgroundColor = UIColor.blue
btnBack.addTarget(self, action: #selector(ImageViewAnimationViewController.btnBackPressed), for: UIControlEvents.touchUpInside)
self.view.addSubview(btnBack)
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
}
func timerEvent(){
// 计算好起始值,结束值
let oldValue = CGFloat(360 / 60 * M_PI / 180 * ClockUseEasingFuncViewController.i)
ClockUseEasingFuncViewController.i += 1
let newValue = CGFloat(360 / 60 * M_PI / 180 * ClockUseEasingFuncViewController.i)
//创建关键帧动画
let keyFrameAnimation: CAKeyframeAnimation = CAKeyframeAnimation()
keyFrameAnimation.keyPath = "transform.rotation.z"
// 设置持续时间
keyFrameAnimation.duration = 0.5
// 设置值
keyFrameAnimation.values = YXEasing.calculateFrame(fromValue: oldValue, toValue: newValue, func: ElasticEaseOut, frameCount: Int(0.5 * 30))
// 每秒增加的角度(设定结果值,在提交动画之前执行)
secLayer.transform = CATransform3DMakeRotation(newValue, 0, 0, 1)
// 提交动画
secLayer.add(keyFrameAnimation, forKey: nil)
}
func btnBackPressed(){
//如果我们启动了一个定时器,在某个界面释放前,将这个定时器停止,甚至置为nil,都不能是这个界面释放,原因是系统的循环池中还保有这个对象。所以我们需要这样做:
if timer.isValid {
// 在官方文档中我们可以看到 [timer invalidate]是唯一的方法将定时器从循环池中移除。
timer.invalidate()
}
timer = nil
self.dismiss(animated: true) {
}
}
func btnStartPressed(){
}
func btnStopPressed(){
}
/*
// MARK: - Navigation
// In a storyboard-based application, you will often want to do a little preparation before navigation
override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
// Get the new view controller using segue.destinationViewController.
// Pass the selected object to the new view controller.
}
*/
}
| mit | a4d5f7871c5c4cb26720e5ee750b8510 | 38.043478 | 156 | 0.663512 | 4.338164 | false | false | false | false |
mshhmzh/firefox-ios | Client/Frontend/Settings/Clearables.swift | 3 | 7109 | /* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/. */
import Foundation
import Shared
import WebKit
import Deferred
import WebImage
private let log = Logger.browserLogger
// Removed Clearables as part of Bug 1226654, but keeping the string around.
private let removedSavedLoginsLabel = NSLocalizedString("Saved Logins", tableName: "ClearPrivateData", comment: "Settings item for clearing passwords and login data")
// A base protocol for something that can be cleared.
protocol Clearable {
func clear() -> Success
var label: String { get }
}
class ClearableError: MaybeErrorType {
private let msg: String
init(msg: String) {
self.msg = msg
}
var description: String { return msg }
}
// Clears our browsing history, including favicons and thumbnails.
class HistoryClearable: Clearable {
let profile: Profile
init(profile: Profile) {
self.profile = profile
}
var label: String {
return NSLocalizedString("Browsing History", tableName: "ClearPrivateData", comment: "Settings item for clearing browsing history")
}
func clear() -> Success {
return profile.history.clearHistory().bind { success in
SDImageCache.sharedImageCache().clearDisk()
SDImageCache.sharedImageCache().clearMemory()
self.profile.recentlyClosedTabs.clearTabs()
NSNotificationCenter.defaultCenter().postNotificationName(NotificationPrivateDataClearedHistory, object: nil)
log.debug("HistoryClearable succeeded: \(success).")
return Deferred(value: success)
}
}
}
struct ClearableErrorType: MaybeErrorType {
let err: ErrorType
init(err: ErrorType) {
self.err = err
}
var description: String {
return "Couldn't clear: \(err)."
}
}
// Clear the web cache. Note, this has to close all open tabs in order to ensure the data
// cached in them isn't flushed to disk.
class CacheClearable: Clearable {
let tabManager: TabManager
init(tabManager: TabManager) {
self.tabManager = tabManager
}
var label: String {
return NSLocalizedString("Cache", tableName: "ClearPrivateData", comment: "Settings item for clearing the cache")
}
func clear() -> Success {
if #available(iOS 9.0, *) {
let dataTypes = Set([WKWebsiteDataTypeDiskCache, WKWebsiteDataTypeMemoryCache])
WKWebsiteDataStore.defaultDataStore().removeDataOfTypes(dataTypes, modifiedSince: NSDate.distantPast(), completionHandler: {})
} else {
// First ensure we close all open tabs first.
tabManager.removeAll()
// Reset the process pool to ensure no cached data is written back
tabManager.resetProcessPool()
// Remove the basic cache.
NSURLCache.sharedURLCache().removeAllCachedResponses()
// Now let's finish up by destroying our Cache directory.
do {
try deleteLibraryFolderContents("Caches")
} catch {
return deferMaybe(ClearableErrorType(err: error))
}
}
log.debug("CacheClearable succeeded.")
return succeed()
}
}
private func deleteLibraryFolderContents(folder: String) throws {
let manager = NSFileManager.defaultManager()
let library = manager.URLsForDirectory(NSSearchPathDirectory.LibraryDirectory, inDomains: .UserDomainMask)[0]
let dir = library.URLByAppendingPathComponent(folder)
let contents = try manager.contentsOfDirectoryAtPath(dir.path!)
for content in contents {
do {
try manager.removeItemAtURL(dir.URLByAppendingPathComponent(content))
} catch where ((error as NSError).userInfo[NSUnderlyingErrorKey] as? NSError)?.code == Int(EPERM) {
// "Not permitted". We ignore this.
log.debug("Couldn't delete some library contents.")
}
}
}
private func deleteLibraryFolder(folder: String) throws {
let manager = NSFileManager.defaultManager()
let library = manager.URLsForDirectory(NSSearchPathDirectory.LibraryDirectory, inDomains: .UserDomainMask)[0]
let dir = library.URLByAppendingPathComponent(folder)
try manager.removeItemAtURL(dir)
}
// Removes all app cache storage.
class SiteDataClearable: Clearable {
let tabManager: TabManager
init(tabManager: TabManager) {
self.tabManager = tabManager
}
var label: String {
return NSLocalizedString("Offline Website Data", tableName: "ClearPrivateData", comment: "Settings item for clearing website data")
}
func clear() -> Success {
if #available(iOS 9.0, *) {
let dataTypes = Set([WKWebsiteDataTypeOfflineWebApplicationCache])
WKWebsiteDataStore.defaultDataStore().removeDataOfTypes(dataTypes, modifiedSince: NSDate.distantPast(), completionHandler: {})
} else {
// First, close all tabs to make sure they don't hold anything in memory.
tabManager.removeAll()
// Then we just wipe the WebKit directory from our Library.
do {
try deleteLibraryFolder("WebKit")
} catch {
return deferMaybe(ClearableErrorType(err: error))
}
}
log.debug("SiteDataClearable succeeded.")
return succeed()
}
}
// Remove all cookies stored by the site. This includes localStorage, sessionStorage, and WebSQL/IndexedDB.
class CookiesClearable: Clearable {
let tabManager: TabManager
init(tabManager: TabManager) {
self.tabManager = tabManager
}
var label: String {
return NSLocalizedString("Cookies", tableName: "ClearPrivateData", comment: "Settings item for clearing cookies")
}
func clear() -> Success {
if #available(iOS 9.0, *) {
let dataTypes = Set([WKWebsiteDataTypeCookies, WKWebsiteDataTypeLocalStorage, WKWebsiteDataTypeSessionStorage, WKWebsiteDataTypeWebSQLDatabases, WKWebsiteDataTypeIndexedDBDatabases])
WKWebsiteDataStore.defaultDataStore().removeDataOfTypes(dataTypes, modifiedSince: NSDate.distantPast(), completionHandler: {})
} else {
// First close all tabs to make sure they aren't holding anything in memory.
tabManager.removeAll()
// Now we wipe the system cookie store (for our app).
let storage = NSHTTPCookieStorage.sharedHTTPCookieStorage()
if let cookies = storage.cookies {
for cookie in cookies {
storage.deleteCookie(cookie)
}
}
// And just to be safe, we also wipe the Cookies directory.
do {
try deleteLibraryFolderContents("Cookies")
} catch {
return deferMaybe(ClearableErrorType(err: error))
}
}
log.debug("CookiesClearable succeeded.")
return succeed()
}
} | mpl-2.0 | 15d1bdef81c4404b182b04e9b041a4db | 35.091371 | 194 | 0.662259 | 5.349135 | false | false | false | false |
hweetty/EasyTransitioning | Example/EasyTransitioning/Example 2/Presented2ViewController.swift | 1 | 1500 | //
// Presented2ViewController.swift
// EasyTransitioning
//
// Created by Jerry Yu on 2016-12-01.
// Copyright © 2016 Jerry Yu. All rights reserved.
//
import UIKit
class Presented2ViewController: UIViewController {
let imageView = UIImageView(image: UIImage(named: "fox"))
override var preferredStatusBarStyle : UIStatusBarStyle {
return .lightContent
}
override func viewDidLoad() {
super.viewDidLoad()
view.backgroundColor = .black
imageView.contentMode = .scaleAspectFit
view.addSubview(imageView)
imageView.translatesAutoresizingMaskIntoConstraints = false
imageView.pinRatio(ratioOfImage(imageView.image!))
NSLayoutConstraint.activate([
imageView.centerXAnchor.constraint(equalTo: view.centerXAnchor),
imageView.centerYAnchor.constraint(equalTo: view.centerYAnchor),
imageView.widthAnchor.constraint(lessThanOrEqualTo: view.widthAnchor),
imageView.heightAnchor.constraint(lessThanOrEqualTo: view.heightAnchor),
])
let gest = UITapGestureRecognizer(target: self, action: #selector(close))
view.addGestureRecognizer(gest)
}
func close() {
self.dismiss(animated: true, completion: nil)
}
func ratioOfImage(_ image: UIImage) -> CGFloat {
return image.size.width / image.size.height
}
}
extension UIView {
func pinRatio(_ widthToHeightRatio: CGFloat) {
NSLayoutConstraint(item: self, attribute: .width, relatedBy: .equal, toItem: self, attribute: .height, multiplier: widthToHeightRatio, constant: 0).isActive = true
}
}
| mit | 6d8b4cc30f222ceb441d93da20c3ef1e | 27.826923 | 165 | 0.75984 | 4.129477 | false | false | false | false |
hhsolar/MemoryMaster-iOS | MemoryMaster/View/CircularCollectionViewCell.swift | 1 | 1449 | //
// CircularCollectionViewCell.swift
// MemoryMaster
//
// Created by apple on 16/10/2017.
// Copyright © 2017 greatwall. All rights reserved.
//
import UIKit
class CircularCollectionViewCell: UICollectionViewCell {
@IBOutlet weak var contentTextView: UITextView!
override func awakeFromNib() {
super.awakeFromNib()
setupUI()
}
private func setupUI() {
contentTextView.layer.cornerRadius = 10
contentTextView.layer.masksToBounds = true
contentTextView.layer.borderWidth = 1
contentTextView.backgroundColor = CustomColor.weakGray
contentTextView.textContainerInset = UIEdgeInsets(top: CustomDistance.midEdge, left: CustomDistance.midEdge, bottom: CustomDistance.midEdge, right: CustomDistance.midEdge)
contentTextView.isEditable = false
contentTextView.isScrollEnabled = false
contentTextView.isUserInteractionEnabled = false
}
func updateUI(content: NSAttributedString)
{
contentTextView.attributedText = content
}
override func apply(_ layoutAttributes: UICollectionViewLayoutAttributes) {
super.apply(layoutAttributes)
let circularlayoutAttributes = layoutAttributes as! CircularCollectionViewLayoutAttributes
self.layer.anchorPoint = circularlayoutAttributes.anchorPoint
self.center.y += (circularlayoutAttributes.anchorPoint.y - 0.5) * self.bounds.height
}
}
| mit | 81e28cb3f845e979f36c4d01b73d8b73 | 33.47619 | 179 | 0.724448 | 5.634241 | false | false | false | false |
Liquidsoul/LocalizationConverter | Sources/LocalizationConverter/Providers/AndroidL10nLanguageFolderProvider.swift | 1 | 2330 | //
// AndroidL10nLanguageFolderProvider.swift
//
// Created by Sébastien Duperron on 26/09/2016.
// Copyright © 2016 Sébastien Duperron
// Released under an MIT license: http://opensource.org/licenses/MIT
//
import Foundation
protocol DirectoryContentProvider {
func contentsOfDirectory(atPath: String) throws -> [String]
}
extension FileManager: DirectoryContentProvider {}
struct AndroidL10nLanguageFolderProvider {
fileprivate let languageToFilePath: [Language: String]
init(folderPath: String, provider: DirectoryContentProvider = FileManager()) throws {
let staticType = type(of: self)
let folders = try staticType.listFolders(atPath: folderPath, provider: provider)
self.languageToFilePath = staticType.listLanguages(from: folders, at: folderPath)
}
private static func listFolders(atPath path: String, provider: DirectoryContentProvider) throws -> [String] {
return try provider.contentsOfDirectory(atPath: path)
}
private static func listLanguages(from folders: [String], at folderPath: String) -> [Language: String] {
let languageToFilePath = folders.reduce([Language: String]()) { (values, folderName) in
guard let language = language(fromFolderName: folderName) else { return values }
let filePath = folderPath
.appending(pathComponent: folderName)
.appending(pathComponent: "strings.xml")
var output = values
output[language] = filePath
return output
}
return languageToFilePath
}
private static func language(fromFolderName folderName: String) -> Language? {
if folderName == "values" { return .base }
let prefix = "values-"
guard folderName.hasPrefix(prefix) else { return nil }
let languageName = folderName.replacingOccurrences(of: prefix, with: "")
return .named(languageName)
}
}
extension AndroidL10nLanguageFolderProvider: LocalizationLanguageProvider {
var languages: [Language] {
return Array(languageToFilePath.keys)
}
func contentProvider(for language: Language) -> LocalizationProvider {
let filePath = languageToFilePath[language]
assert(filePath != nil)
return AndroidL10nFileProvider(filePath: filePath!)
}
}
| mit | b17345e5f3410e827f1d4cca187a7dbb | 36.532258 | 113 | 0.694886 | 4.868201 | false | false | false | false |
SuperAwesomeLTD/sa-mobile-sdk-ios-demo | AwesomeAdsDemo/LoadAdsTask.swift | 1 | 2829 | //
// LoadAdsTask.swift
// AwesomeAdsDemo
//
// Created by Gabriel Coman on 10/10/2017.
// Copyright © 2017 SuperAwesome. All rights reserved.
//
import UIKit
import RxSwift
import SuperAwesome
struct ApprovalsFilter : Codable {
let approved : String
let placements : PlacementFilter
init(approved: Bool, placements: PlacementFilter) {
self.approved = String(approved)
self.placements = placements
}
}
struct PlacementFilter : Codable {
let like : String
init(like: Int) {
self.like = "%" + String(like) + "%"
}
}
class LoadAdsTask: Task {
typealias Input = LoadAdsRequest
typealias Output = DemoAd
typealias Result = Observable<DemoAd>
func execute(withInput input: LoadAdsRequest) -> Observable<DemoAd> {
let url = "https://api.dashboard.superawesome.tv/v2/company/\(input.company)/approvals"
let filter = ApprovalsFilter(
approved: true,
placements: PlacementFilter(
like: input.placementId
)
)
let encoder = JSONEncoder()
encoder.keyEncodingStrategy = .approvalsFilterStrategy
let filterData = try? encoder.encode(filter)
let filterString = String(data: filterData!, encoding: .utf8)!.addingPercentEncoding(withAllowedCharacters: .alphanumerics)!
let query:[String : String] = [
"country" : "all",
"scope" : "liveAds",
"countryInclude" : "true",
"limit" : "500",
"offset" : "0",
"filter" : filterString
]
let header:[String : Any] = [
"aa-user-token": input.token
]
let network = SANetwork()
return Observable.create { subscriber -> Disposable in
network.sendGET(url, withQuery: query, andHeader: header) { (status, payload, success) in
var results: [DemoAd] = []
if let payload = payload, let data = payload.data(using: .utf8) {
do {
results = try JSONDecoder().decode(DemoAdList.self, from: data).data
} catch {
subscriber.onError(AAError.NoInternet)
}
} else {
subscriber.onError(AAError.NoInternet)
}
if (results.count == 0) {
subscriber.onError(AAError.ParseError)
}
else {
for result in results {
subscriber.onNext(result)
}
subscriber.onCompleted()
}
}
return Disposables.create()
}
}
}
| apache-2.0 | 7df8aaff9a7843ab8db17337ce0aa991 | 27.565657 | 132 | 0.524399 | 4.859107 | false | false | false | false |
Neku/GEOSwift | GEOSwift/MapboxGL/MapboxGLAnnotations.swift | 4 | 5341 | //
// MapboxGLAnnotations.swift
//
// Created by Andrea Cremaschi on 26/05/15.
// Copyright (c) 2015 andreacremaschi. All rights reserved.
//
import Foundation
import Mapbox
import MapKit
// MARK: - MGLShape creation convenience function
public protocol GEOSwiftMapboxGL {
/**
A convenience method to create a `MGLShape` ready to be added to a `MKMapView`.
:returns: A MGLShape representing this geometry.
*/
func mapboxShape() -> MGLShape
}
extension Geometry : GEOSwiftMapboxGL {
public func mapboxShape() -> MGLShape {
if let geom = self as? MultiPolygon {
let geometryCollectionOverlay = MGLShapesCollection(geometryCollection: geom)
return geometryCollectionOverlay
} else
if let geom = self as? MultiLineString {
let geometryCollectionOverlay = MGLShapesCollection(geometryCollection: geom)
return geometryCollectionOverlay
} else
if let geom = self as? MultiPoint {
let geometryCollectionOverlay = MGLShapesCollection(geometryCollection: geom)
return geometryCollectionOverlay
} else
if let geom = self as? GeometryCollection {
let geometryCollectionOverlay = MGLShapesCollection(geometryCollection: geom)
return geometryCollectionOverlay
}
// this method is just a workaround for limited extension capabilities in Swift 1.2
// and should NEVER actually return MGLShape()
return MGLShape()
}
}
extension Waypoint : GEOSwiftMapboxGL {
override public func mapboxShape() -> MGLShape {
let pointAnno = MGLPointAnnotation()
pointAnno.coordinate = CLLocationCoordinateFromCoordinate(self.coordinate)
return pointAnno
}
}
extension LineString : GEOSwiftMapboxGL {
override public func mapboxShape() -> MGLShape {
let pointAnno: MGLPolyline = MGLPolyline()
var coordinates = self.points.map({ (point: Coordinate) ->
CLLocationCoordinate2D in
return CLLocationCoordinateFromCoordinate(point)
})
var polyline = MGLPolyline(coordinates: &coordinates,
count: UInt(coordinates.count))
return polyline
}
}
extension Polygon : GEOSwiftMapboxGL {
override public func mapboxShape() -> MGLShape {
var exteriorRingCoordinates = self.exteriorRing.points.map({ (point: Coordinate) ->
CLLocationCoordinate2D in
return CLLocationCoordinateFromCoordinate(point)
})
let interiorRings = self.interiorRings.map({ (linearRing: LinearRing) ->
MGLPolygon in
return MGLPolygonWithCoordinatesSequence(linearRing.points)
})
let polygon = MGLPolygon(coordinates: &exteriorRingCoordinates, count: UInt(exteriorRingCoordinates.count)/*, interiorPolygons: interiorRings*/)
return polygon
}
}
// TODO: restore this in Swift 2.0
//extension GeometryCollection : GEOSwiftMapKit {
// override public func mapboxShape() -> MGLShape {
// let geometryCollectionOverlay = MGLShapesCollection(geometryCollection: self as! GeometryCollection<Geometry>)
// return geometryCollectionOverlay
// }
//}
private func MGLPolygonWithCoordinatesSequence(coordinates: CoordinatesCollection) -> MGLPolygon {
var coordinates = coordinates.map({ (point: Coordinate) ->
CLLocationCoordinate2D in
return CLLocationCoordinateFromCoordinate(point)
})
return MGLPolygon(coordinates: &coordinates,
count: UInt(coordinates.count))
}
/**
MGLShape subclass for GeometryCollections.
The property `shapes` contains MGLShape subclasses instances. When drawing shapes on a map be careful to the fact that that these shapes could be overlays OR annotations.
*/
public class MGLShapesCollection : MGLShape, MGLAnnotation, MGLOverlay {
let shapes: Array<MGLShape>
public let centroid: CLLocationCoordinate2D
public let overlayBounds: MGLCoordinateBounds
required public init<T>(geometryCollection: GeometryCollection<T>) {
let shapes = geometryCollection.geometries.map({ (geometry: T) ->
MGLShape in
return geometry.mapboxShape()
})
self.centroid = CLLocationCoordinateFromCoordinate(geometryCollection.centroid().coordinate)
self.shapes = shapes
if let envelope = geometryCollection.envelope() as? Polygon {
let exteriorRing = envelope.exteriorRing
let sw = CLLocationCoordinateFromCoordinate(exteriorRing.points[0])
let ne = CLLocationCoordinateFromCoordinate(exteriorRing.points[2])
self.overlayBounds = MGLCoordinateBounds(sw:sw, ne:ne)
} else {
let zeroCoord = CLLocationCoordinate2DMake(0, 0)
self.overlayBounds = MGLCoordinateBounds(sw:zeroCoord, ne:zeroCoord)
}
}
override public var coordinate: CLLocationCoordinate2D { get {
return centroid
}}
// TODO: implement using "intersect" method (actually it seems that mapboxgl never calls it...)
public func intersectsOverlayBounds(overlayBounds: MGLCoordinateBounds) -> Bool {
return true
}
}
| mit | 96536f148787eb072ffaa0b80925667f | 36.879433 | 170 | 0.67684 | 5.557752 | false | false | false | false |
zdrzdr/QEDSwift | Pods/Kingfisher/Sources/UIButton+Kingfisher.swift | 2 | 29533 | //
// UIButton+Kingfisher.swift
// Kingfisher
//
// Created by Wei Wang on 15/4/13.
//
// Copyright (c) 2016 Wei Wang <[email protected]>
//
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in
// all copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
// THE SOFTWARE.
#if os(iOS) || os(tvOS)
import UIKit
/**
* Set image to use from web for a specified state.
*/
extension UIButton {
/**
Set an image to use for a specified state with a resource.
It will ask for Kingfisher's manager to get the image for the `cacheKey` property in `resource` and then set it for a button state.
The memory and disk will be searched first. If the manager does not find it, it will try to download the image at the `resource.downloadURL` and store it with `resource.cacheKey` for next use.
- parameter resource: Resource object contains information such as `cacheKey` and `downloadURL`.
- parameter state: The state that uses the specified image.
- returns: A task represents the retrieving process.
*/
public func kf_setImageWithResource(resource: Resource,
forState state: UIControlState) -> RetrieveImageTask
{
return kf_setImageWithResource(resource, forState: state, placeholderImage: nil, optionsInfo: nil, progressBlock: nil, completionHandler: nil)
}
/**
Set an image to use for a specified state with a URL.
It will ask for Kingfisher's manager to get the image for the URL and then set it for a button state.
The memory and disk will be searched first with `URL.absoluteString` as the cache key. If the manager does not find it, it will try to download the image at this URL and store the image with `URL.absoluteString` as cache key for next use.
If you need to specify the key other than `URL.absoluteString`, please use resource version of these APIs with `resource.cacheKey` set to what you want.
- parameter URL: The URL of image for specified state.
- parameter state: The state that uses the specified image.
- returns: A task represents the retrieving process.
*/
public func kf_setImageWithURL(URL: NSURL,
forState state: UIControlState) -> RetrieveImageTask
{
return kf_setImageWithURL(URL, forState: state, placeholderImage: nil, optionsInfo: nil, progressBlock: nil, completionHandler: nil)
}
/**
Set an image to use for a specified state with a resource and a placeholder image.
- parameter resource: Resource object contains information such as `cacheKey` and `downloadURL`.
- parameter state: The state that uses the specified image.
- parameter placeholderImage: A placeholder image when retrieving the image at URL.
- returns: A task represents the retrieving process.
*/
public func kf_setImageWithResource(resource: Resource,
forState state: UIControlState,
placeholderImage: UIImage?) -> RetrieveImageTask
{
return kf_setImageWithResource(resource, forState: state, placeholderImage: placeholderImage, optionsInfo: nil, progressBlock: nil, completionHandler: nil)
}
/**
Set an image to use for a specified state with a URL and a placeholder image.
- parameter URL: The URL of image for specified state.
- parameter state: The state that uses the specified image.
- parameter placeholderImage: A placeholder image when retrieving the image at URL.
- returns: A task represents the retrieving process.
*/
public func kf_setImageWithURL(URL: NSURL,
forState state: UIControlState,
placeholderImage: UIImage?) -> RetrieveImageTask
{
return kf_setImageWithURL(URL, forState: state, placeholderImage: placeholderImage, optionsInfo: nil, progressBlock: nil, completionHandler: nil)
}
/**
Set an image to use for a specified state with a resource, a placeholder image and options.
- parameter resource: Resource object contains information such as `cacheKey` and `downloadURL`.
- parameter state: The state that uses the specified image.
- parameter placeholderImage: A placeholder image when retrieving the image at URL.
- parameter optionsInfo: A dictionary could control some behaviors. See `KingfisherOptionsInfo` for more.
- returns: A task represents the retrieving process.
*/
public func kf_setImageWithResource(resource: Resource,
forState state: UIControlState,
placeholderImage: UIImage?,
optionsInfo: KingfisherOptionsInfo?) -> RetrieveImageTask
{
return kf_setImageWithResource(resource, forState: state, placeholderImage: placeholderImage, optionsInfo: optionsInfo, progressBlock: nil, completionHandler: nil)
}
/**
Set an image to use for a specified state with a URL, a placeholder image and options.
- parameter URL: The URL of image for specified state.
- parameter state: The state that uses the specified image.
- parameter placeholderImage: A placeholder image when retrieving the image at URL.
- parameter optionsInfo: A dictionary could control some behaviors. See `KingfisherOptionsInfo` for more.
- returns: A task represents the retrieving process.
*/
public func kf_setImageWithURL(URL: NSURL,
forState state: UIControlState,
placeholderImage: UIImage?,
optionsInfo: KingfisherOptionsInfo?) -> RetrieveImageTask
{
return kf_setImageWithURL(URL, forState: state, placeholderImage: placeholderImage, optionsInfo: optionsInfo, progressBlock: nil, completionHandler: nil)
}
/**
Set an image to use for a specified state with a resource, a placeholder image, options and completion handler.
- parameter resource: Resource object contains information such as `cacheKey` and `downloadURL`.
- parameter state: The state that uses the specified image.
- parameter placeholderImage: A placeholder image when retrieving the image at URL.
- parameter optionsInfo: A dictionary could control some behaviors. See `KingfisherOptionsInfo` for more.
- parameter completionHandler: Called when the image retrieved and set.
- returns: A task represents the retrieving process.
*/
public func kf_setImageWithResource(resource: Resource,
forState state: UIControlState,
placeholderImage: UIImage?,
optionsInfo: KingfisherOptionsInfo?,
completionHandler: CompletionHandler?) -> RetrieveImageTask
{
return kf_setImageWithResource(resource, forState: state, placeholderImage: placeholderImage, optionsInfo: optionsInfo, progressBlock: nil, completionHandler: completionHandler)
}
/**
Set an image to use for a specified state with a URL, a placeholder image, options and completion handler.
- parameter URL: The URL of image for specified state.
- parameter state: The state that uses the specified image.
- parameter placeholderImage: A placeholder image when retrieving the image at URL.
- parameter optionsInfo: A dictionary could control some behaviors. See `KingfisherOptionsInfo` for more.
- parameter completionHandler: Called when the image retrieved and set.
- returns: A task represents the retrieving process.
*/
public func kf_setImageWithURL(URL: NSURL,
forState state: UIControlState,
placeholderImage: UIImage?,
optionsInfo: KingfisherOptionsInfo?,
completionHandler: CompletionHandler?) -> RetrieveImageTask
{
return kf_setImageWithURL(URL, forState: state, placeholderImage: placeholderImage, optionsInfo: optionsInfo, progressBlock: nil, completionHandler: completionHandler)
}
/**
Set an image to use for a specified state with a resource, a placeholder image, options, progress handler and completion handler.
- parameter resource: Resource object contains information such as `cacheKey` and `downloadURL`.
- parameter state: The state that uses the specified image.
- parameter placeholderImage: A placeholder image when retrieving the image at URL.
- parameter optionsInfo: A dictionary could control some behaviors. See `KingfisherOptionsInfo` for more.
- parameter progressBlock: Called when the image downloading progress gets updated.
- parameter completionHandler: Called when the image retrieved and set.
- returns: A task represents the retrieving process.
*/
public func kf_setImageWithResource(resource: Resource,
forState state: UIControlState,
placeholderImage: UIImage?,
optionsInfo: KingfisherOptionsInfo?,
progressBlock: DownloadProgressBlock?,
completionHandler: CompletionHandler?) -> RetrieveImageTask
{
setImage(placeholderImage, forState: state)
kf_setWebURL(resource.downloadURL, forState: state)
let task = KingfisherManager.sharedManager.retrieveImageWithResource(resource, optionsInfo: optionsInfo,
progressBlock: { receivedSize, totalSize in
if let progressBlock = progressBlock {
dispatch_async(dispatch_get_main_queue(), { () -> Void in
progressBlock(receivedSize: receivedSize, totalSize: totalSize)
})
}
},
completionHandler: {[weak self] image, error, cacheType, imageURL in
dispatch_async_safely_main_queue {
if let sSelf = self {
sSelf.kf_setImageTask(nil)
if imageURL == sSelf.kf_webURLForState(state) && image != nil {
sSelf.setImage(image, forState: state)
}
completionHandler?(image: image, error: error, cacheType: cacheType, imageURL: imageURL)
}
}
})
kf_setImageTask(task)
return task
}
/**
Set an image to use for a specified state with a URL, a placeholder image, options, progress handler and completion handler.
- parameter URL: The URL of image for specified state.
- parameter state: The state that uses the specified image.
- parameter placeholderImage: A placeholder image when retrieving the image at URL.
- parameter optionsInfo: A dictionary could control some behaviors. See `KingfisherOptionsInfo` for more.
- parameter progressBlock: Called when the image downloading progress gets updated.
- parameter completionHandler: Called when the image retrieved and set.
- returns: A task represents the retrieving process.
*/
public func kf_setImageWithURL(URL: NSURL,
forState state: UIControlState,
placeholderImage: UIImage?,
optionsInfo: KingfisherOptionsInfo?,
progressBlock: DownloadProgressBlock?,
completionHandler: CompletionHandler?) -> RetrieveImageTask
{
return kf_setImageWithResource(Resource(downloadURL: URL),
forState: state,
placeholderImage: placeholderImage,
optionsInfo: optionsInfo,
progressBlock: progressBlock,
completionHandler: completionHandler)
}
}
private var lastURLKey: Void?
private var imageTaskKey: Void?
// MARK: - Runtime for UIButton image
extension UIButton {
/**
Get the image URL binded to this button for a specified state.
- parameter state: The state that uses the specified image.
- returns: Current URL for image.
*/
public func kf_webURLForState(state: UIControlState) -> NSURL? {
return kf_webURLs[NSNumber(unsignedLong:state.rawValue)] as? NSURL
}
private func kf_setWebURL(URL: NSURL, forState state: UIControlState) {
kf_webURLs[NSNumber(unsignedLong:state.rawValue)] = URL
}
private var kf_webURLs: NSMutableDictionary {
var dictionary = objc_getAssociatedObject(self, &lastURLKey) as? NSMutableDictionary
if dictionary == nil {
dictionary = NSMutableDictionary()
kf_setWebURLs(dictionary!)
}
return dictionary!
}
private func kf_setWebURLs(URLs: NSMutableDictionary) {
objc_setAssociatedObject(self, &lastURLKey, URLs, .OBJC_ASSOCIATION_RETAIN_NONATOMIC)
}
private var kf_imageTask: RetrieveImageTask? {
return objc_getAssociatedObject(self, &imageTaskKey) as? RetrieveImageTask
}
private func kf_setImageTask(task: RetrieveImageTask?) {
objc_setAssociatedObject(self, &imageTaskKey, task, .OBJC_ASSOCIATION_RETAIN_NONATOMIC)
}
}
/**
* Set background image to use from web for a specified state.
*/
extension UIButton {
/**
Set the background image to use for a specified state with a resource.
It will ask for Kingfisher's manager to get the image for the `cacheKey` property in `resource` and then set it for a button state.
The memory and disk will be searched first. If the manager does not find it, it will try to download the image at the `resource.downloadURL` and store it with `resource.cacheKey` for next use.
- parameter resource: Resource object contains information such as `cacheKey` and `downloadURL`.
- parameter state: The state that uses the specified image.
- returns: A task represents the retrieving process.
*/
public func kf_setBackgroundImageWithResource(resource: Resource,
forState state: UIControlState) -> RetrieveImageTask
{
return kf_setBackgroundImageWithResource(resource, forState: state, placeholderImage: nil, optionsInfo: nil, progressBlock: nil, completionHandler: nil)
}
/**
Set the background image to use for a specified state with a URL.
It will ask for Kingfisher's manager to get the image for the URL and then set it for a button state.
The memory and disk will be searched first with `URL.absoluteString` as the cache key. If the manager does not find it, it will try to download the image at this URL and store the image with `URL.absoluteString` as cache key for next use.
If you need to specify the key other than `URL.absoluteString`, please use resource version of these APIs with `resource.cacheKey` set to what you want.
- parameter URL: The URL of image for specified state.
- parameter state: The state that uses the specified image.
- returns: A task represents the retrieving process.
*/
public func kf_setBackgroundImageWithURL(URL: NSURL,
forState state: UIControlState) -> RetrieveImageTask
{
return kf_setBackgroundImageWithURL(URL, forState: state, placeholderImage: nil, optionsInfo: nil, progressBlock: nil, completionHandler: nil)
}
/**
Set the background image to use for a specified state with a resource and a placeholder image.
- parameter resource: Resource object contains information such as `cacheKey` and `downloadURL`.
- parameter state: The state that uses the specified image.
- parameter placeholderImage: A placeholder image when retrieving the image at URL.
- returns: A task represents the retrieving process.
*/
public func kf_setBackgroundImageWithResource(resource: Resource,
forState state: UIControlState,
placeholderImage: UIImage?) -> RetrieveImageTask
{
return kf_setBackgroundImageWithResource(resource, forState: state, placeholderImage: placeholderImage, optionsInfo: nil, progressBlock: nil, completionHandler: nil)
}
/**
Set the background image to use for a specified state with a URL and a placeholder image.
- parameter URL: The URL of image for specified state.
- parameter state: The state that uses the specified image.
- parameter placeholderImage: A placeholder image when retrieving the image at URL.
- returns: A task represents the retrieving process.
*/
public func kf_setBackgroundImageWithURL(URL: NSURL,
forState state: UIControlState,
placeholderImage: UIImage?) -> RetrieveImageTask
{
return kf_setBackgroundImageWithURL(URL, forState: state, placeholderImage: placeholderImage, optionsInfo: nil, progressBlock: nil, completionHandler: nil)
}
/**
Set the background image to use for a specified state with a resource, a placeholder image and options.
- parameter resource: Resource object contains information such as `cacheKey` and `downloadURL`.
- parameter state: The state that uses the specified image.
- parameter placeholderImage: A placeholder image when retrieving the image at URL.
- parameter optionsInfo: A dictionary could control some behaviors. See `KingfisherOptionsInfo` for more.
- returns: A task represents the retrieving process.
*/
public func kf_setBackgroundImageWithResource(resource: Resource,
forState state: UIControlState,
placeholderImage: UIImage?,
optionsInfo: KingfisherOptionsInfo?) -> RetrieveImageTask
{
return kf_setBackgroundImageWithResource(resource, forState: state, placeholderImage: placeholderImage, optionsInfo: optionsInfo, progressBlock: nil, completionHandler: nil)
}
/**
Set the background image to use for a specified state with a URL, a placeholder image and options.
- parameter URL: The URL of image for specified state.
- parameter state: The state that uses the specified image.
- parameter placeholderImage: A placeholder image when retrieving the image at URL.
- parameter optionsInfo: A dictionary could control some behaviors. See `KingfisherOptionsInfo` for more.
- returns: A task represents the retrieving process.
*/
public func kf_setBackgroundImageWithURL(URL: NSURL,
forState state: UIControlState,
placeholderImage: UIImage?,
optionsInfo: KingfisherOptionsInfo?) -> RetrieveImageTask
{
return kf_setBackgroundImageWithURL(URL, forState: state, placeholderImage: placeholderImage, optionsInfo: optionsInfo, progressBlock: nil, completionHandler: nil)
}
/**
Set the background image to use for a specified state with a resource, a placeholder image, options and completion handler.
- parameter resource: Resource object contains information such as `cacheKey` and `downloadURL`.
- parameter state: The state that uses the specified image.
- parameter placeholderImage: A placeholder image when retrieving the image at URL.
- parameter optionsInfo: A dictionary could control some behaviors. See `KingfisherOptionsInfo` for more.
- parameter completionHandler: Called when the image retrieved and set.
- returns: A task represents the retrieving process.
*/
public func kf_setBackgroundImageWithResource(resource: Resource,
forState state: UIControlState,
placeholderImage: UIImage?,
optionsInfo: KingfisherOptionsInfo?,
completionHandler: CompletionHandler?) -> RetrieveImageTask
{
return kf_setBackgroundImageWithResource(resource, forState: state, placeholderImage: placeholderImage, optionsInfo: optionsInfo, progressBlock: nil, completionHandler: completionHandler)
}
/**
Set the background image to use for a specified state with a URL, a placeholder image, options and completion handler.
- parameter URL: The URL of image for specified state.
- parameter state: The state that uses the specified image.
- parameter placeholderImage: A placeholder image when retrieving the image at URL.
- parameter optionsInfo: A dictionary could control some behaviors. See `KingfisherOptionsInfo` for more.
- parameter completionHandler: Called when the image retrieved and set.
- returns: A task represents the retrieving process.
*/
public func kf_setBackgroundImageWithURL(URL: NSURL,
forState state: UIControlState,
placeholderImage: UIImage?,
optionsInfo: KingfisherOptionsInfo?,
completionHandler: CompletionHandler?) -> RetrieveImageTask
{
return kf_setBackgroundImageWithURL(URL, forState: state, placeholderImage: placeholderImage, optionsInfo: optionsInfo, progressBlock: nil, completionHandler: completionHandler)
}
/**
Set the background image to use for a specified state with a resource,
a placeholder image, options progress handler and completion handler.
- parameter resource: Resource object contains information such as `cacheKey` and `downloadURL`.
- parameter state: The state that uses the specified image.
- parameter placeholderImage: A placeholder image when retrieving the image at URL.
- parameter optionsInfo: A dictionary could control some behaviors. See `KingfisherOptionsInfo` for more.
- parameter progressBlock: Called when the image downloading progress gets updated.
- parameter completionHandler: Called when the image retrieved and set.
- returns: A task represents the retrieving process.
*/
public func kf_setBackgroundImageWithResource(resource: Resource,
forState state: UIControlState,
placeholderImage: UIImage?,
optionsInfo: KingfisherOptionsInfo?,
progressBlock: DownloadProgressBlock?,
completionHandler: CompletionHandler?) -> RetrieveImageTask
{
setBackgroundImage(placeholderImage, forState: state)
kf_setBackgroundWebURL(resource.downloadURL, forState: state)
let task = KingfisherManager.sharedManager.retrieveImageWithResource(resource, optionsInfo: optionsInfo,
progressBlock: { receivedSize, totalSize in
if let progressBlock = progressBlock {
dispatch_async(dispatch_get_main_queue(), { () -> Void in
progressBlock(receivedSize: receivedSize, totalSize: totalSize)
})
}
},
completionHandler: { [weak self] image, error, cacheType, imageURL in
dispatch_async_safely_main_queue {
if let sSelf = self {
sSelf.kf_setBackgroundImageTask(nil)
if imageURL == sSelf.kf_backgroundWebURLForState(state) && image != nil {
sSelf.setBackgroundImage(image, forState: state)
}
completionHandler?(image: image, error: error, cacheType: cacheType, imageURL: imageURL)
}
}
})
kf_setBackgroundImageTask(task)
return task
}
/**
Set the background image to use for a specified state with a URL,
a placeholder image, options progress handler and completion handler.
- parameter URL: The URL of image for specified state.
- parameter state: The state that uses the specified image.
- parameter placeholderImage: A placeholder image when retrieving the image at URL.
- parameter optionsInfo: A dictionary could control some behaviors. See `KingfisherOptionsInfo` for more.
- parameter progressBlock: Called when the image downloading progress gets updated.
- parameter completionHandler: Called when the image retrieved and set.
- returns: A task represents the retrieving process.
*/
public func kf_setBackgroundImageWithURL(URL: NSURL,
forState state: UIControlState,
placeholderImage: UIImage?,
optionsInfo: KingfisherOptionsInfo?,
progressBlock: DownloadProgressBlock?,
completionHandler: CompletionHandler?) -> RetrieveImageTask
{
return kf_setBackgroundImageWithResource(Resource(downloadURL: URL),
forState: state,
placeholderImage: placeholderImage,
optionsInfo: optionsInfo,
progressBlock: progressBlock,
completionHandler: completionHandler)
}
}
private var lastBackgroundURLKey: Void?
private var backgroundImageTaskKey: Void?
// MARK: - Runtime for UIButton background image
extension UIButton {
/**
Get the background image URL binded to this button for a specified state.
- parameter state: The state that uses the specified background image.
- returns: Current URL for background image.
*/
public func kf_backgroundWebURLForState(state: UIControlState) -> NSURL? {
return kf_backgroundWebURLs[NSNumber(unsignedLong:state.rawValue)] as? NSURL
}
private func kf_setBackgroundWebURL(URL: NSURL, forState state: UIControlState) {
kf_backgroundWebURLs[NSNumber(unsignedLong:state.rawValue)] = URL
}
private var kf_backgroundWebURLs: NSMutableDictionary {
var dictionary = objc_getAssociatedObject(self, &lastBackgroundURLKey) as? NSMutableDictionary
if dictionary == nil {
dictionary = NSMutableDictionary()
kf_setBackgroundWebURLs(dictionary!)
}
return dictionary!
}
private func kf_setBackgroundWebURLs(URLs: NSMutableDictionary) {
objc_setAssociatedObject(self, &lastBackgroundURLKey, URLs, .OBJC_ASSOCIATION_RETAIN_NONATOMIC)
}
private var kf_backgroundImageTask: RetrieveImageTask? {
return objc_getAssociatedObject(self, &backgroundImageTaskKey) as? RetrieveImageTask
}
private func kf_setBackgroundImageTask(task: RetrieveImageTask?) {
objc_setAssociatedObject(self, &backgroundImageTaskKey, task, .OBJC_ASSOCIATION_RETAIN_NONATOMIC)
}
}
// MARK: - Cancel image download tasks.
extension UIButton {
/**
Cancel the image download task bounded to the image view if it is running.
Nothing will happen if the downloading has already finished.
*/
public func kf_cancelImageDownloadTask() {
kf_imageTask?.downloadTask?.cancel()
}
/**
Cancel the background image download task bounded to the image view if it is running.
Nothing will happen if the downloading has already finished.
*/
public func kf_cancelBackgroundImageDownloadTask() {
kf_backgroundImageTask?.downloadTask?.cancel()
}
}
#elseif os(OSX)
import AppKit
extension NSButton {
// Not Implemented yet.
}
#endif
| mit | 058fe86e6460f2ae4e8f4cec862bb45d | 49.140917 | 242 | 0.649815 | 5.897165 | false | false | false | false |
flowsprenger/RxLifx-Swift | RxLifxApi/RxLifxApi/LightsGroupLocationService.swift | 1 | 8148 | /*
Copyright 2018 Florian Sprenger
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated
documentation files (the "Software"), to deal in the Software without restriction, including without limitation the
rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit
persons to whom the Software is furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all copies or substantial portions of the
Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE
WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR
COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*/
import Foundation
import LifxDomain
public class LightsGroup {
public let identifier: String
public let location: LightsLocation
public private(set) var lights: [Light] = []
init(identifier: String, location: LightsLocation) {
self.identifier = identifier
self.location = location
}
public var label: String {
return lights.reduce((Date(timeIntervalSince1970: 0), "")) { (result, light: Light) in
if let group = light.group.value {
if (result.0 > group.updatedAt) {
return result
} else {
return (group.updatedAt, group.label)
}
} else {
return result
}
}.1
}
fileprivate func add(light: Light) {
lights.append(light)
}
fileprivate func remove(light: Light) {
if let index = lights.firstIndex(of: light) {
lights.remove(at: index)
}
}
}
public class LightsLocation {
fileprivate var groupsById: [String: LightsGroup] = [:]
public let identifier: String
public var groups: Dictionary<String, LightsGroup>.Values {
get {
return groupsById.values
}
}
init(identifier: String) {
self.identifier = identifier
}
public var label: String {
return groupsById.flatMap { (_, group) in
group.lights
}.reduce((Date(timeIntervalSince1970: 0), "")) { (result, light: Light) in
if let location = light.location.value {
if (result.0 > location.updatedAt) {
return result
} else {
return (location.updatedAt, location.label)
}
} else {
return result
}
}.1
}
fileprivate func add(group: LightsGroup) {
groupsById[group.identifier] = group
}
fileprivate func remove(group: LightsGroup) {
groupsById.removeValue(forKey: group.identifier)
}
}
public protocol GroupLocationChangeDispatcher {
func groupAdded(group: LightsGroup)
func groupRemoved(group: LightsGroup)
func groupChanged(group: LightsGroup)
func locationAdded(location: LightsLocation)
func locationRemoved(location: LightsLocation)
func locationChanged(location: LightsLocation)
}
public class LightsGroupLocationService: LightsChangeDispatcher, LightServiceExtension {
private var locationsById: [String: LightsLocation] = [:]
private let lightsChangeDispatcher: LightsChangeDispatcher
private var groupLocationChangeDispatcher: GroupLocationChangeDispatcher?
public var locations: Dictionary<String, LightsLocation>.Values {
get{
return locationsById.values
}
}
public required init(wrappedChangeDispatcher: LightsChangeDispatcher) {
self.lightsChangeDispatcher = wrappedChangeDispatcher
}
public func setListener( groupLocationChangeDispatcher: GroupLocationChangeDispatcher?){
self.groupLocationChangeDispatcher = groupLocationChangeDispatcher
}
public func start(source: LightSource) {
}
public func stop() {
locationsById = [:]
}
public func groupOf(light: Light) -> LightsGroup? {
return locationsById[light.location.value?.identifier ?? ""]?.groupsById[light.group.value?.identifier ?? ""]
}
public func locationOf(light: Light) -> LightsLocation? {
return locationsById[light.location.value?.identifier ?? ""]
}
public func notifyChange(light: Light, property: LightPropertyName, oldValue: Any?, newValue: Any?) {
switch (property) {
case LightPropertyName.group:
if let locationId = light.location.value?.identifier, let groupId = (oldValue as? LightGroup)?.identifier, let location = locationsById[locationId], let group = location.groupsById[groupId] {
removeLightFromLocationGroup(light: light, location: location, group: group)
}
addLightToLocationAndGroup(light: light, location: light.location.value, group: newValue as? LightGroup)
break;
case LightPropertyName.location:
if let locationId = (oldValue as? LightLocation)?.identifier, let groupId = light.location.value?.identifier, let location = locationsById[locationId], let group = location.groupsById[groupId] {
removeLightFromLocationGroup(light: light, location: location, group: group)
}
addLightToLocationAndGroup(light: light, location: newValue as? LightLocation, group: light.group.value )
break;
default:
break;
}
lightsChangeDispatcher.notifyChange(light: light, property: property, oldValue: oldValue, newValue: newValue)
}
public func lightAdded(light: Light) {
addLightToLocationAndGroup(light: light, location: light.location.value, group: light.group.value)
lightsChangeDispatcher.lightAdded(light: light)
}
private func removeLightFromLocationGroup(light: Light, location: LightsLocation, group: LightsGroup) {
group.remove(light: light)
groupLocationChangeDispatcher?.groupChanged(group: group)
if (group.lights.count == 0) {
removeGroupFrom(location: location, group: group)
}
if (location.groupsById.count == 0) {
remove(location: location)
}
}
private func addLightToLocationAndGroup(light: Light, location: LightLocation?, group: LightGroup?) {
if let locationId = location?.identifier, let groupId = group?.identifier {
let location: LightsLocation = locationsById[locationId] ?? add(location: LightsLocation(identifier: locationId))
let group: LightsGroup = location.groupsById[groupId] ?? addGroupTo(location: location, group: LightsGroup(identifier: groupId, location: location))
group.add(light: light)
groupLocationChangeDispatcher?.groupChanged(group: group)
groupLocationChangeDispatcher?.locationChanged(location: location)
}
}
private func addGroupTo(location: LightsLocation, group: LightsGroup) -> LightsGroup {
location.add(group: group)
groupLocationChangeDispatcher?.groupAdded(group: group)
groupLocationChangeDispatcher?.locationChanged(location: location)
return group
}
private func removeGroupFrom(location: LightsLocation, group: LightsGroup) {
location.remove(group: group)
groupLocationChangeDispatcher?.groupRemoved(group: group)
}
private func add(location: LightsLocation) -> LightsLocation {
locationsById[location.identifier] = location
groupLocationChangeDispatcher?.locationAdded(location: location)
return location
}
private func remove(location: LightsLocation) {
locationsById.removeValue(forKey: location.identifier)
groupLocationChangeDispatcher?.locationRemoved(location: location)
}
}
| mit | b962a9e3665c470dd1e301b1c23ca046 | 36.897674 | 206 | 0.678081 | 5.166772 | false | false | false | false |
AlexMcArdle/LooseFoot | LooseFoot/LayoutExampleNode+Layouts.swift | 1 | 3065 |
import AsyncDisplayKit
extension HeaderWithRightAndLeftItems {
override func layoutSpecThatFits(_ constrainedSize: ASSizeRange) -> ASLayoutSpec {
let nameLocationStack = ASStackLayoutSpec.vertical()
nameLocationStack.style.flexShrink = 1.0
nameLocationStack.style.flexGrow = 1.0
if postLocationNode.attributedText != nil {
nameLocationStack.children = [userNameNode, postLocationNode]
} else {
nameLocationStack.children = [userNameNode]
}
let headerStackSpec = ASStackLayoutSpec(direction: .horizontal,
spacing: 40,
justifyContent: .start,
alignItems: .center,
children: [nameLocationStack, postTimeNode])
return ASInsetLayoutSpec(insets: UIEdgeInsets(top: 0, left: 10, bottom: 0, right: 10), child: headerStackSpec)
}
}
extension PhotoWithInsetTextOverlay {
override func layoutSpecThatFits(_ constrainedSize: ASSizeRange) -> ASLayoutSpec {
let photoDimension: CGFloat = constrainedSize.max.width / 4.0
photoNode.style.preferredSize = CGSize(width: photoDimension, height: photoDimension)
// INFINITY is used to make the inset unbounded
let insets = UIEdgeInsets(top: CGFloat.infinity, left: 12, bottom: 12, right: 12)
let textInsetSpec = ASInsetLayoutSpec(insets: insets, child: titleNode)
return ASOverlayLayoutSpec(child: photoNode, overlay: textInsetSpec)
}
}
extension PhotoWithOutsetIconOverlay {
override func layoutSpecThatFits(_ constrainedSize: ASSizeRange) -> ASLayoutSpec {
iconNode.style.preferredSize = CGSize(width: 40, height: 40);
iconNode.style.layoutPosition = CGPoint(x: 150, y: 0);
photoNode.style.preferredSize = CGSize(width: 150, height: 150);
photoNode.style.layoutPosition = CGPoint(x: 40 / 2.0, y: 40 / 2.0);
let absoluteSpec = ASAbsoluteLayoutSpec(children: [photoNode, iconNode])
// ASAbsoluteLayoutSpec's .sizing property recreates the behavior of ASDK Layout API 1.0's "ASStaticLayoutSpec"
absoluteSpec.sizing = .sizeToFit
return absoluteSpec;
}
}
extension FlexibleSeparatorSurroundingContent {
override func layoutSpecThatFits(_ constrainedSize: ASSizeRange) -> ASLayoutSpec {
topSeparator.style.flexGrow = 1.0
bottomSeparator.style.flexGrow = 1.0
textNode.style.alignSelf = .center
let verticalStackSpec = ASStackLayoutSpec.vertical()
verticalStackSpec.spacing = 20
verticalStackSpec.justifyContent = .center
verticalStackSpec.children = [topSeparator, textNode, bottomSeparator]
return ASInsetLayoutSpec(insets:UIEdgeInsets(top: 60, left: 0, bottom: 60, right: 0), child: verticalStackSpec)
}
}
| gpl-3.0 | 9ef69c0da623afb1b9fb2236454fd3cb | 38.294872 | 119 | 0.638173 | 5.302768 | false | false | false | false |
artursDerkintis/Starfly | Starfly/ParallaxView.swift | 1 | 4068 | //
// ParallaxView.swift
// YouTube
//
// Created by Arturs Derkintis on 12/31/15.
// Copyright © 2015 Starfly. All rights reserved.
//
import UIKit
let hidableViewTag = 7175
class ParallaxView: UIView, UIGestureRecognizerDelegate {
var timer : NSTimer?
dynamic var parallax = false
var longRec : UILongPressGestureRecognizer!
override init(frame: CGRect) {
super.init(frame: frame)
longRec = UILongPressGestureRecognizer(target: self, action: "long:")
longRec.minimumPressDuration = 0.3
addGestureRecognizer(longRec)
}
func long(sender : UILongPressGestureRecognizer){
if sender.state == .Began{
allowParallax()
}else if sender.state == .Changed{
if parallax{
let point = sender.locationInView(self)
if CGRectContainsPoint(self.superview!.frame, point){
let newX = point.x - (bounds.width / 2)
let newY = abs(point.y - (bounds.height / 2))
layer.zPosition = 100
var rotationAndPerspectiveTransform = CATransform3DIdentity;
rotationAndPerspectiveTransform.m34 = 1.0 / -500;
rotationAndPerspectiveTransform = CATransform3DRotate(rotationAndPerspectiveTransform,
(-newX / 7) * CGFloat(M_PI) / 180.0,
-newY / 7,
10.0,
0.0)
UIView.animateWithDuration(0.3) { () -> Void in
self.layer.transform = rotationAndPerspectiveTransform
}
}else{
endParallax()
}
}
}else if sender.state == .Ended{
endParallax()
}
}
func pan(panGesture : UIPanGestureRecognizer){
switch panGesture.state{
case .Began:
longRec.enabled = false
break
case .Changed:
if parallax{
let point = panGesture.locationInView(self)
if CGRectContainsPoint(self.superview!.frame, point){
let newX = point.x - bounds.width / 2
var newY = point.y - bounds.height / 2
if newY < 0{
newY = newY * -1
}
layer.zPosition = 100
var rotationAndPerspectiveTransform = CATransform3DIdentity;
rotationAndPerspectiveTransform.m34 = 1.0 / -500;
rotationAndPerspectiveTransform = CATransform3DRotate(rotationAndPerspectiveTransform,
(-newX / 5) * CGFloat(M_PI) / 180.0,
-newY / 5,
4.0,
0.0)
UIView.animateWithDuration(0.3) { () -> Void in
self.layer.transform = rotationAndPerspectiveTransform
}
}else{
endParallax()
}
}
break
case .Ended, .Failed, .Cancelled:
endParallax()
break
default:
break
}
}
func allowParallax(){
parallax = true
UIView.animateWithDuration(0.3) { () -> Void in
self.setAlphaForViews(0.0)
}
}
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
func endParallax(){
timer?.invalidate()
UIView.animateWithDuration(0.3) { () -> Void in
self.setAlphaForViews(1.0)
self.layer.transform = CATransform3DIdentity
}
parallax = false
longRec.enabled = true
}
func setAlphaForViews(alpha : CGFloat){
for subview in subviews{
if subview.tag == hidableViewTag{
subview.alpha = alpha
}
}
}
}
| mit | 54f92ceabf0254aa9ff3d1e8306e16ed | 31.536 | 106 | 0.499877 | 5.533333 | false | false | false | false |
kean/Nuke | Sources/Nuke/Prefetching/ImagePrefetcher.swift | 1 | 7962 | // The MIT License (MIT)
//
// Copyright (c) 2015-2022 Alexander Grebenyuk (github.com/kean).
import Foundation
/// Prefetches and caches images to eliminate delays when requesting the same
/// images later.
///
/// The prefetcher cancels all of the outstanding tasks when deallocated.
///
/// All ``ImagePrefetcher`` methods are thread-safe and are optimized to be used
/// even from the main thread during scrolling.
public final class ImagePrefetcher: @unchecked Sendable {
private let pipeline: ImagePipeline
private var tasks = [ImageLoadKey: Task]()
private let destination: Destination
let queue = OperationQueue() // internal for testing
public var didComplete: (() -> Void)? // called when # of in-flight tasks decrements to 0
/// Pauses the prefetching.
///
/// - note: When you pause, the prefetcher will finish outstanding tasks
/// (by default, there are only 2 at a time), and pause the rest.
public var isPaused: Bool = false {
didSet { queue.isSuspended = isPaused }
}
/// The priority of the requests. By default, ``ImageRequest/Priority-swift.enum/low``.
///
/// Changing the priority also changes the priority of all of the outstanding
/// tasks managed by the prefetcher.
public var priority: ImageRequest.Priority = .low {
didSet {
let newValue = priority
pipeline.queue.async { self.didUpdatePriority(to: newValue) }
}
}
private var _priority: ImageRequest.Priority = .low
/// Prefetching destination.
public enum Destination: Sendable {
/// Prefetches the image and stores it in both the memory and the disk
/// cache (make sure to enable it).
case memoryCache
/// Prefetches the image data and stores it in disk caches. It does not
/// require decoding the image data and therefore requires less CPU.
///
/// - important: This option is incompatible with ``ImagePipeline/DataCachePolicy/automatic``
/// (for requests with processors) and ``ImagePipeline/DataCachePolicy/storeEncodedImages``.
case diskCache
}
/// Initializes the ``ImagePrefetcher`` instance.
///
/// - parameters:
/// - pipeline: The pipeline used for loading images.
/// - destination: By default load images in all cache layers.
/// - maxConcurrentRequestCount: 2 by default.
public init(pipeline: ImagePipeline = ImagePipeline.shared,
destination: Destination = .memoryCache,
maxConcurrentRequestCount: Int = 2) {
self.pipeline = pipeline
self.destination = destination
self.queue.maxConcurrentOperationCount = maxConcurrentRequestCount
self.queue.underlyingQueue = pipeline.queue
#if TRACK_ALLOCATIONS
Allocations.increment("ImagePrefetcher")
#endif
}
deinit {
let tasks = self.tasks.values // Make sure we don't retain self
pipeline.queue.async {
for task in tasks {
task.cancel()
}
}
#if TRACK_ALLOCATIONS
Allocations.decrement("ImagePrefetcher")
#endif
}
/// Starts prefetching images for the given URL.
///
/// See also ``startPrefetching(with:)-718dg`` that works with ``ImageRequest``.
public func startPrefetching(with urls: [URL]) {
startPrefetching(with: urls.map { ImageRequest(url: $0) })
}
/// Starts prefetching images for the given requests.
///
/// When you need to display the same image later, use the ``ImagePipeline``
/// or the view extensions to load it as usual. The pipeline will take care
/// of coalescing the requests to avoid any duplicate work.
///
/// The priority of the requests is set to the priority of the prefetcher
/// (`.low` by default).
///
/// See also ``startPrefetching(with:)-1jef2`` that works with `URL`.
public func startPrefetching(with requests: [ImageRequest]) {
pipeline.queue.async {
for request in requests {
var request = request
if self._priority != request.priority {
request.priority = self._priority
}
self._startPrefetching(with: request)
}
}
}
private func _startPrefetching(with request: ImageRequest) {
guard pipeline.cache[request] == nil else {
return // The image is already in memory cache
}
let key = request.makeImageLoadKey()
guard tasks[key] == nil else {
return // Already started prefetching
}
let task = Task(request: request, key: key)
task.operation = queue.add { [weak self] finish in
guard let self = self else { return finish() }
self.loadImage(task: task, finish: finish)
}
tasks[key] = task
}
private func loadImage(task: Task, finish: @escaping () -> Void) {
switch destination {
case .diskCache:
task.imageTask = pipeline.loadData(with: task.request, isConfined: true, queue: pipeline.queue, progress: nil) { [weak self] _ in
self?._remove(task)
finish()
}
case .memoryCache:
task.imageTask = pipeline.loadImage(with: task.request, isConfined: true, queue: pipeline.queue, progress: nil) { [weak self] _ in
self?._remove(task)
finish()
}
}
task.onCancelled = finish
}
private func _remove(_ task: Task) {
guard tasks[task.key] === task else { return } // Should never happen
tasks[task.key] = nil
if tasks.isEmpty {
didComplete?()
}
}
/// Stops prefetching images for the given URLs and cancels outstanding
/// requests.
///
/// See also ``stopPrefetching(with:)-8cdam`` that works with ``ImageRequest``.
public func stopPrefetching(with urls: [URL]) {
stopPrefetching(with: urls.map { ImageRequest(url: $0) })
}
/// Stops prefetching images for the given requests and cancels outstanding
/// requests.
///
/// You don't need to balance the number of `start` and `stop` requests.
/// If you have multiple screens with prefetching, create multiple instances
/// of ``ImagePrefetcher``.
///
/// See also ``stopPrefetching(with:)-2tcyq`` that works with `URL`.
public func stopPrefetching(with requests: [ImageRequest]) {
pipeline.queue.async {
for request in requests {
self._stopPrefetching(with: request)
}
}
}
private func _stopPrefetching(with request: ImageRequest) {
if let task = tasks.removeValue(forKey: request.makeImageLoadKey()) {
task.cancel()
}
}
/// Stops all prefetching tasks.
public func stopPrefetching() {
pipeline.queue.async {
self.tasks.values.forEach { $0.cancel() }
self.tasks.removeAll()
}
}
private func didUpdatePriority(to priority: ImageRequest.Priority) {
guard _priority != priority else { return }
_priority = priority
for task in tasks.values {
task.imageTask?.priority = priority
}
}
private final class Task: @unchecked Sendable {
let key: ImageLoadKey
let request: ImageRequest
weak var imageTask: ImageTask?
weak var operation: Operation?
var onCancelled: (() -> Void)?
init(request: ImageRequest, key: ImageLoadKey) {
self.request = request
self.key = key
}
// When task is cancelled, it is removed from the prefetcher and can
// never get cancelled twice.
func cancel() {
operation?.cancel()
imageTask?.cancel()
onCancelled?()
}
}
}
| mit | edbfd75abf8efbe655a2f021d0ddf67e | 34.544643 | 142 | 0.609771 | 4.896679 | false | false | false | false |
butterproject/butter-ios | Butter/UI/Views/TablePickerView.swift | 1 | 8465 | //
// TablePickerView.swift
// Butter
//
// Created by Moorice on 12-10-15.
// Copyright © 2015 Butter Project. All rights reserved.
//
import UIKit
@objc public protocol TablePickerViewDelegate {
optional func tablePickerView(tablePickerView: TablePickerView, didSelect item: String)
optional func tablePickerView(tablePickerView: TablePickerView, didDeselect item: String)
optional func tablePickerView(tablePickerView: TablePickerView, didClose items: [String])
optional func tablePickerView(tablePickerView: TablePickerView, willClose items: [String])
optional func tablePickerView(tablePickerView: TablePickerView, didChange items: [String])
}
public class TablePickerView: UIView, UITableViewDataSource, UITableViewDelegate {
@IBOutlet private var view: UIView!
@IBOutlet public weak var tableView: UITableView!
@IBOutlet public weak var toolbar: UIToolbar!
@IBOutlet public weak var button: UIBarButtonItem!
public var delegate : TablePickerViewDelegate?
private var superView : UIView?
private var dataSourceKeys = [String]()
private var dataSourceValues = [String]()
private (set) public var _selectedItems = [String]()
private var cellBackgroundColor : UIColor?
private var cellBackgroundColorSelected : UIColor?
private var cellTextColor : UIColor?
private var multipleSelect : Bool = true
private var nullAllowed : Bool = true
private var speed : Double = 0.2
// MARK: Init methods
public init(superView: UIView) {
super.init(frame: CGRectZero)
self.superView = superView
prepareView()
}
public init(superView: UIView, sourceDict: [String : String]?, _ delegate: TablePickerViewDelegate?) {
super.init(frame: CGRectZero)
self.superView = superView
if let sourceDict = sourceDict {
self.setSourceDictionay(sourceDict)
}
self.delegate = delegate
prepareView()
}
public init(superView: UIView, sourceArray: [String]?, _ delegate: TablePickerViewDelegate?) {
super.init(frame: CGRectZero)
self.superView = superView
if let sourceArray = sourceArray {
self.setSourceArray(sourceArray)
}
self.delegate = delegate
prepareView()
}
required public init?(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)
prepareView()
}
// MARK: Set source and get selectted items
public func setSourceDictionay(source : [String : String]) {
let sortedKeysAndValues = source.sort({ $0.1 < $1.1 })
for (key, value) in sortedKeysAndValues {
self.dataSourceKeys.append(key)
self.dataSourceValues.append(value)
}
tableView?.reloadData()
}
public func setSourceArray(source : [String]) {
self.dataSourceKeys = source
self.dataSourceValues = source
tableView?.reloadData()
}
public func setSelected(items : [String]) {
_selectedItems = items
tableView?.reloadData()
}
public func setMultipleSelect(multiple : Bool) {
multipleSelect = multiple
}
public func setNullAllowed(multiple : Bool) {
nullAllowed = multiple
}
public func deselect(item : String) {
if let index = _selectedItems.indexOf(item) {
_selectedItems.removeAtIndex(index)
tableView?.reloadData()
delegate?.tablePickerView?(self, didDeselect: item)
}
}
public func deselectButThis(item : String) {
for _item in _selectedItems {
if _item != item {
delegate?.tablePickerView?(self, didDeselect: item)
}
}
_selectedItems = [item]
tableView?.reloadData()
}
public func selectedItems() -> [String] {
return _selectedItems
}
// MARK: Style TablePickerView
// You can also style this view directly since the IBOutlets are public
public func setButtonText(text : String) {
button.title = text
}
public func setToolBarBackground(color : UIColor) {
toolbar.backgroundColor = color
}
public func setCellBackgroundColor(color : UIColor) {
cellBackgroundColor = color
}
public func setCellBackgroundColorSelected(color : UIColor) {
cellBackgroundColorSelected = color
}
public func setCellTextColor(color : UIColor) {
cellTextColor = color
}
public func setCellSeperatorColor(color : UIColor) {
self.tableView.separatorColor = color
}
public func setAnimationSpeed(speed : Double) {
self.speed = speed
}
// MARK: Show / hide view
public func show() {
if let superView = superView {
self.hidden = false
var newFrame = self.frame
newFrame.origin.y = superView.frame.height - self.frame.height
UIView.animateWithDuration(speed, delay: 0, options: .CurveEaseInOut, animations: { () -> Void in
self.frame = newFrame
}, completion: { (finished) in })
}
}
public func hide() {
if isVisibe() {
if let superView = superView {
var newFrame = self.frame
newFrame.origin.y = superView.frame.height
delegate?.tablePickerView?(self, willClose: selectedItems())
UIView.animateWithDuration(speed, delay: 0, options: .CurveEaseInOut, animations: { () -> Void in
self.frame = newFrame
}, completion: { (finished) in
self.hidden = true
self.delegate?.tablePickerView?(self, didClose: self.selectedItems())
})
}
}
}
public func toggle() {
if isVisibe() {
hide()
} else {
show()
}
}
public func isVisibe() -> Bool {
return !self.hidden
}
// MARK: UITabelView
public func numberOfSectionsInTableView(tableView: UITableView) -> Int {
return 1
}
public func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return dataSourceKeys.count
}
public func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
let cell = UITableViewCell()
cell.textLabel?.text = dataSourceValues[indexPath.row]
if let cellBackgroundColor = cellBackgroundColor {
cell.backgroundColor = cellBackgroundColor
}
if let cellBackgroundColorSelected = cellBackgroundColorSelected {
let bg = UIView()
bg.backgroundColor = cellBackgroundColorSelected
cell.selectedBackgroundView = bg
} else {
let blurEffectView = UIVisualEffectView(effect: UIBlurEffect(style: .Light))
cell.selectedBackgroundView = blurEffectView
}
if let cellTextColor = cellTextColor {
cell.textLabel?.textColor = cellTextColor
cell.tintColor = cellTextColor
}
if _selectedItems.contains(dataSourceKeys[indexPath.row]) {
cell.accessoryType = .Checkmark
} else {
cell.accessoryType = .None
}
return cell
}
public func tableView(tableView: UITableView, didSelectRowAtIndexPath indexPath: NSIndexPath) {
let cell = tableView.cellForRowAtIndexPath(indexPath)!
let selectedItem = dataSourceKeys[indexPath.row]
if _selectedItems.contains(selectedItem) && (nullAllowed || _selectedItems.count > 1) {
_selectedItems.removeAtIndex(_selectedItems.indexOf(selectedItem)!)
delegate?.tablePickerView?(self, didDeselect: selectedItem)
delegate?.tablePickerView?(self, didChange: _selectedItems)
cell.accessoryType = .None
} else {
if !multipleSelect && _selectedItems.count > 0 {
let oldSelected = _selectedItems[0]
_selectedItems = []
if let index = dataSourceKeys.indexOf(oldSelected) {
let oldCell = tableView.cellForRowAtIndexPath(NSIndexPath(forItem: index, inSection: 0))
oldCell?.accessoryType = .None
}
}
_selectedItems.append(selectedItem)
cell.accessoryType = .Checkmark
delegate?.tablePickerView?(self, didSelect: selectedItem)
delegate?.tablePickerView?(self, didChange: _selectedItems)
}
tableView.deselectRowAtIndexPath(indexPath, animated: true)
}
// MARK: Private methods
private func prepareView() {
loadNib()
tableView.tableFooterView = UIView.init(frame: CGRectZero)
let borderTop = CALayer()
borderTop.frame = CGRectMake(0.0, toolbar.frame.height - 1, toolbar.frame.width, 0.5);
borderTop.backgroundColor = UIColor(red:0.17, green:0.17, blue:0.17, alpha:1.0).CGColor
toolbar.layer.addSublayer(borderTop)
if let superView = superView {
self.hidden = true
let height = superView.frame.height / 2.7
let frameSelf = CGRect(x: 0, y: superView.frame.height, width: superView.frame.width, height: height)
var framePicker = frameSelf
framePicker.origin.y = 0
self.frame = frameSelf
self.view.frame = framePicker
}
}
private func loadNib() {
UINib(nibName: "TablePickerView", bundle: nil).instantiateWithOwner(self, options: nil)[0] as? UIView
self.addSubview(self.view)
}
@IBAction func done(sender: AnyObject) {
hide()
}
}
| gpl-3.0 | 4316c44fd4c0df73d47e0d49956bb19a | 26.391586 | 113 | 0.722353 | 3.98869 | false | false | false | false |
rushabh55/NaviTag-iOS | iOS/SwiftExample/MapViewController_BACKUP_6257.swift | 1 | 5722 |
import UIKit
import MapKit
import CoreLocation
import Foundation
class MapViewController: UIViewController, MKMapViewDelegate, CLLocationManagerDelegate, NSURLConnectionDelegate {
@IBOutlet var mapView : MKMapView?
var data = NSMutableData()
override init(nibName nibNameOrNil: String?, bundle nibBundleOrNil: NSBundle?) {
super.init(nibName: nibNameOrNil, bundle: nibBundleOrNil)
// Custom initialization
}
required init(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)
}
override func viewWillAppear(animated: Bool) {
super.viewWillAppear(animated)
startConnection()
}
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
self.mapView?.mapType = MKMapType.Hybrid
// self.mapView.delegate = self
let location = "1 Lomb Memorial Drive, Rochester, NY 14623"
var geocoder:CLGeocoder = CLGeocoder()
geocoder.geocodeAddressString(location, {(placemarks, error) -> Void in
if((error) != nil){
println("Error", error)
}
else if let placemark = placemarks?[0] as? CLPlacemark {
var placemark:CLPlacemark = placemarks[0] as CLPlacemark
var coordinates:CLLocationCoordinate2D = placemark.location.coordinate
var pointAnnotation:MKPointAnnotation = MKPointAnnotation()
pointAnnotation.coordinate = coordinates
pointAnnotation.title = "RIT"
self.mapView?.addAnnotation(pointAnnotation)
self.mapView?.centerCoordinate = coordinates
self.mapView?.selectAnnotation(pointAnnotation, animated: true)
self.mapView?.zoomEnabled = true
let mapCoord = coordinates
var mapCamera = MKMapCamera(lookingAtCenterCoordinate: mapCoord, fromEyeCoordinate: mapCoord, eyeAltitude: 1000)
self.mapView?.setCamera(mapCamera, animated: true)
println("Added annotation to map view")
}
})
}
func startConnection(){
let urlPath: String = "http://rushg.me/TreasureHunt/hunt.php?q=getNearestHunts&coord=number,number"
var url: NSURL = NSURL(string: urlPath)!
var request: NSURLRequest = NSURLRequest(URL: url)
var connection: NSURLConnection = NSURLConnection(request: request, delegate: self, startImmediately: false)!
connection.start()
}
func connection(connection: NSURLConnection!, didReceiveData data: NSData!){
self.data.appendData(data)
}
func buttonAction(sender: UIButton!){
startConnection()
}
func connectionDidFinishLoading(connection: NSURLConnection!) {
var err: NSError
// throwing an error on the line below (can't figure out where the error message is)
var jsonResult: NSDictionary = NSJSONSerialization.JSONObjectWithData(data, options: NSJSONReadingOptions.MutableContainers, error: nil) as NSDictionary
// var aArray: AvailableHunts = AvailableHunts(JSONString: jsonResult.description)
// println(aArray.name1) // Output is "myUser"
var arrayObjects: AnyObject? = jsonResult.valueForKey("status")
<<<<<<< HEAD
//let zero = arrayObjects[1]
=======
// let zero = arrayObjects[1]
>>>>>>> c3ef6f36f163cfc68e72c9efc217580772197d34
println(arrayObjects)
}
// http://rushg.me/TreasureHunt/hunt.php?q=getNearestHunts&coord=number,number
func mapView (mapView: MKMapView!,
viewForAnnotation annotation: MKAnnotation!) -> MKAnnotationView! {
var pinView:MKPinAnnotationView = MKPinAnnotationView()
pinView.annotation = annotation
pinView.pinColor = MKPinAnnotationColor.Red
pinView.animatesDrop = true
pinView.canShowCallout = true
return pinView
}
func mapView(mapView: MKMapView!,
didSelectAnnotationView view: MKAnnotationView!){
println("Selected annotation")
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
class AvailableHunts : NSObject {
var name1 : String = ""
var name2 : String = ""
var name3 : String = ""
var name4 : String = ""
var name5 : String = ""
var name6 : String = ""
var name7 : String = ""
var name8 : String = ""
var name9 : String = ""
init(JSONString: String) {
super.init()
var error : NSError?
let JSONData = JSONString.dataUsingEncoding(NSUTF8StringEncoding, allowLossyConversion: false)
let JSONDictionary: Dictionary = NSJSONSerialization.JSONObjectWithData(JSONData!, options: nil, error: &error) as NSDictionary
// Loop
for (key, value) in JSONDictionary {
let keyName = key as String
let keyValue: String = value as String
// If property exists
if (self.respondsToSelector(NSSelectorFromString(keyName))) {
self.setValue(keyValue, forKey: keyName)
}
}
// Or you can do it with using
// self.setValuesForKeysWithDictionary(JSONDictionary)
// instead of loop method above
}
}
}
| apache-2.0 | fbde639473afb54225b5e14e890dc132 | 36.398693 | 160 | 0.607305 | 5.51784 | false | false | false | false |
raphweiner/SwiftDrop | SwiftDropExample/SwiftDropExample/SwiftDropExample/ViewControllerWithSwiftDrop.swift | 1 | 1464 | //
// ViewControllerWithSwiftDrop.swift
// SwiftDropExample
//
// Created by Raphael Weiner on 12/26/14.
// Copyright (c) 2014 raphaelweiner. All rights reserved.
//
import UIKit
class ViewControllerWithSwiftDrop: UIViewController, SwiftDropDelegate {
var swiftDrop: SwiftDrop!
var viewControllerOne: UIViewController!
var viewControllerTwo: UIViewController!
override func viewDidLoad() {
super.viewDidLoad()
view.backgroundColor = UIColor.greenColor()
viewControllerOne = UIViewController()
viewControllerOne.view.backgroundColor = UIColor.blueColor()
viewControllerOne.title = "Blue View Controller"
viewControllerTwo = UIViewController()
viewControllerTwo.view.backgroundColor = UIColor.redColor()
viewControllerTwo.title = "Red View Controller"
swiftDrop = SwiftDrop()
swiftDrop.menuItems = [viewControllerOne.title!, viewControllerTwo.title!]
swiftDrop.navigationController = navigationController
swiftDrop.delegate = self
}
func didSelectItemAt(index: Int) {
var selectedViewController: UIViewController?
switch index {
case 0:
selectedViewController = viewControllerOne
case 1:
selectedViewController = viewControllerTwo
default:
break
}
navigationController!.pushViewController(selectedViewController!, animated: true)
}
}
| mit | 309a6a00190932b7972371926bae2d0b | 27.705882 | 89 | 0.693306 | 5.809524 | false | false | false | false |
ivanbruel/SwipeIt | SwipeIt/ViewControllers/Onboarding/WalkthroughViewController.swift | 1 | 1882 | //
// WalkthroughViewController.swift
// Reddit
//
// Created by Ivan Bruel on 25/04/16.
// Copyright © 2016 Faber Ventures. All rights reserved.
//
import UIKit
import Localizable
// MARK: Properties and Lifecycle
class WalkthroughViewController: UIViewController {
var viewModel: WalkthroughViewModel! = WalkthroughViewModel()
@IBOutlet private weak var loginButton: UIButton! {
didSet {
loginButton.setTitle(tr(.WalkthroughButtonLogin), forState: .Normal)
}
}
override func viewDidLoad() {
super.viewDidLoad()
setup()
}
}
// MARK: Setup
extension WalkthroughViewController {
private func setup() {
viewModel.loginResult
.bindNext { [weak self] error in
guard let error = error else {
self?.goToMainStoryboard()
return
}
self?.showLoginError(error)
}.addDisposableTo(self.rx_disposeBag)
}
}
// MARK: UI
extension WalkthroughViewController: AlerteableViewController {
private func goToMainStoryboard() {
performSegue(StoryboardSegue.Onboarding.Main)
}
private func showLoginError(error: ErrorType) {
let loginError = error as? LoginError ?? .Unknown
presentAlert(tr(.LoginErrorTitle), message: loginError.description,
buttonTitle: tr(.AlertButtonOK))
}
}
// MARK: Segue
extension WalkthroughViewController {
override func prepareForSegue(segue: UIStoryboardSegue, sender: AnyObject?) {
guard let rootViewController = segue.navigationRootViewController else {
return
}
switch rootViewController {
case let loginViewController as LoginViewController:
loginViewController.viewModel = viewModel.loginViewModel
case let subscriptionsViewController as SubscriptionsViewController:
subscriptionsViewController.viewModel = viewModel.subscriptionsViewModel
default:
return
}
}
}
| mit | 69ea76ab9cac5dd52f20818afcf8d939 | 22.810127 | 79 | 0.715045 | 5.125341 | false | false | false | false |
jlecomte/EarthquakeTracker | EarthquakeTracker/MapViewController.swift | 1 | 4612 | //
// MapViewController.swift
// EarthquakeTracker
//
// Created by Julien Lecomte on 10/11/14.
// Copyright (c) 2014 Julien Lecomte. All rights reserved.
//
import UIKit
import MapKit
import CoreLocation
class EarthquakePointAnnotation: MKPointAnnotation {
var earthquake: Earthquake!
}
class MapViewController: UIViewController, CLLocationManagerDelegate, MKMapViewDelegate {
@IBOutlet weak var map: MKMapView!
var locmgr: CLLocationManager!
var annotations: [MKPointAnnotation] = []
override func viewDidLoad() {
super.viewDidLoad()
// Setup location manager...
locmgr = CLLocationManager()
locmgr.delegate = self
locmgr.desiredAccuracy = kCLLocationAccuracyBest
if (locmgr.respondsToSelector(Selector("requestWhenInUseAuthorization"))) {
locmgr.requestWhenInUseAuthorization()
}
locmgr.startUpdatingLocation()
// Setup map view...
map.delegate = self
map.showsUserLocation = true
}
override func viewDidAppear(animated: Bool) {
// In case you just changed your settings...
refreshMapAnnotations()
}
func locationManager(manager: CLLocationManager!, didUpdateLocations locations: [AnyObject]!) {
// We only need the user's location once, so stop updating location immediately!
locmgr.stopUpdatingLocation()
var location = locations[0] as CLLocation
var region = MKCoordinateRegionMakeWithDistance(location.coordinate, 100000, 100000)
map.setRegion(region, animated: true)
}
func mapView(mapView: MKMapView!, regionDidChangeAnimated animated: Bool) {
refreshMapAnnotations()
}
func refreshMapAnnotations() {
// This trivial implementation removes all existing annotations,
// requests the USGS service, and creates new annotations based
// on the data returned whenever the user moves the map or zooms
// in or out. Needless to say that it is highly inefficient...
let client = USGSClient.sharedInstance
client.setRegion(map.region.center, maxradius: max(map.region.span.latitudeDelta, map.region.span.longitudeDelta))
client.getEarthquakeList() {
(earthquakes: [Earthquake]!, error: NSError!) -> Void in
if error != nil {
var alert = UIAlertController(title: "Error", message: error.description, preferredStyle: UIAlertControllerStyle.Alert)
self.presentViewController(alert, animated: false, completion: nil)
} else {
// This copies the array...
var oldAnnotations = self.map.annotations
// First add new annotations...
for earthquake in earthquakes {
var epa = EarthquakePointAnnotation()
epa.earthquake = earthquake
epa.coordinate = CLLocationCoordinate2D(
latitude: earthquake.latitude as CLLocationDegrees!,
longitude: earthquake.longitude as CLLocationDegrees!)
let dateFormatter = NSDateFormatter()
dateFormatter.dateFormat = "M/d 'at' h:m a"
let time = dateFormatter.stringFromDate(earthquake.time!)
epa.title = "Mag. \(earthquake.magnitude!) event on \(time)"
epa.subtitle = earthquake.place
self.map.addAnnotation(epa)
}
// ... and only remove the old ones to prevent flickering!
self.map.removeAnnotations(oldAnnotations)
}
}
}
func mapView(mapView: MKMapView!, viewForAnnotation annotation: MKAnnotation!) -> MKAnnotationView! {
if !annotation.isKindOfClass(EarthquakePointAnnotation) {
return nil
}
var epa = annotation as EarthquakePointAnnotation
var v = map.dequeueReusableAnnotationViewWithIdentifier("EarthquakePin") as MKPinAnnotationView!
if v == nil {
v = MKPinAnnotationView(annotation: epa, reuseIdentifier: "EarthquakePin")
}
v.canShowCallout = true
if epa.earthquake.magnitude < 2.5 {
v.image = UIImage(named: "map_pin_green")
} else if epa.earthquake.magnitude < 3.5 {
v.image = UIImage(named: "map_pin_yellow")
} else if epa.earthquake.magnitude < 5 {
v.image = UIImage(named: "map_pin_orange")
} else {
v.image = UIImage(named: "map_pin_red")
}
return v
}
}
| mit | 64065f302c7f2f098d44536b93b8f85d | 34.206107 | 135 | 0.627927 | 5.38785 | false | false | false | false |
JLCdeSwift/DangTangDemo | DanTangdemo/Classes/Me(我)/Model/LCSettings.swift | 1 | 672 | //
// LCSettings.swift
// DanTangdemo
//
// Created by 冀柳冲 on 2017/7/6.
// Copyright © 2017年 sunny冲哥. All rights reserved.
//
import UIKit
class LCSettings: NSObject {
var iconImage: String?
var leftTitle: String?
var rightTitle: String?
var isHiddenSwitch: Bool?
var isHiddenRightTip: Bool?
init(dict: [String: AnyObject]) {
super.init()
iconImage = dict["iconImage"] as? String
leftTitle = dict["leftTitle"] as? String
rightTitle = dict["rightTitle"] as? String
isHiddenSwitch = dict["isHiddenSwitch"] as? Bool
isHiddenRightTip = dict["isHiddenRightTip"] as? Bool
}
}
| mit | e73effd1ce297b320a3192df7f2f9647 | 22.535714 | 60 | 0.638847 | 3.809249 | false | false | false | false |
naru-jpn/pencil | Sources/Pencil.swift | 1 | 2302 | //
// Vendor.swift
// PencilExample
//
// Created by naru on 2016/09/21.
// Copyright © 2016年 naru. All rights reserved.
//
import Foundation
public protocol ReadWrite: Writable, Readable { }
open class Pencil {
open class func read<T: ReadWriteElement>(_ data: Data) -> T? {
guard let components: Components = T.devide(data: data) else {
return nil
}
return T.read(from: components)
}
open class func read<T: ReadWriteElement>(_ data: Data) -> [T]? {
guard let array: [Data] = [T].devide(data: data) else {
return nil
}
return [T].read(array)
}
open class func read<T: ReadWriteElement>(_ data: Data) -> Set<T>? {
guard let array: [Data] = Set<T>.devide(data: data) else {
return nil
}
return Set<T>.read(array)
}
open class func read<T: ReadWriteElement>(_ data: Data) -> [String: T]? {
guard let dictionary: [String: Data] = [String: T].devide(data: data) else {
return nil
}
return [String: T].read(dictionary)
}
open class func read<T: CustomReadWriteElement>(_ data: Data) -> T? {
guard let components: Components = T.devide(data: data) else {
return nil
}
return T.read(from: components)
}
open class func read<T: CustomReadWriteElement>(_ data: Data) -> [T]? {
guard let array: [Data] = [T].devide(data: data) else {
return nil
}
return [T].read(array)
}
open class func read<T: CustomReadWriteElement>(_ data: Data) -> Set<T>? {
guard let array: [Data] = Set<T>.devide(data: data) else {
return nil
}
return Set<T>.read(array)
}
open class func read<T: CustomReadWriteElement>(_ data: Data) -> [String: T]? {
guard let dictionary: [String: Data] = [String: T].devide(data: data) else {
return nil
}
return [String: T].read(dictionary)
}
open class func read<T: RawRepresentable>(_ data: Data) -> T? where T.RawValue: ReadWriteElement {
guard let components: Components = T.RawValue.devide(data: data) else {
return nil
}
return T.read(from: components)
}
}
| mit | bc957fec71562fd7444511527bf6fea7 | 28.474359 | 102 | 0.563723 | 3.857383 | false | false | false | false |
blstream/AugmentedSzczecin_iOS | AugmentedSzczecin/AugmentedSzczecin/UIViewController+Keyboard.swift | 1 | 3493 | //
// UIViewController+Keyboard.swift
//
// Created by Håkon Bogen on 10/12/14.
// Copyright (c) 2014 Håkon Bogen. All rights reserved.
// MIT LICENSE
import UIKit
private var scrollViewKey : UInt8 = 0
extension UIViewController {
public func setupKeyboardNotifcationListenerForScrollView(scrollView: UIScrollView) {
NSNotificationCenter.defaultCenter().addObserver(self, selector: Selector("keyboardWillShow:"), name: UIKeyboardWillShowNotification, object: nil)
NSNotificationCenter.defaultCenter().addObserver(self, selector: Selector("keyboardWillHide:"), name: UIKeyboardWillHideNotification, object: nil)
internalScrollView = scrollView
}
public func removeKeyboardNotificationListeners() {
NSNotificationCenter.defaultCenter().removeObserver(self, name: UIKeyboardWillShowNotification, object: nil)
NSNotificationCenter.defaultCenter().removeObserver(self, name: UIKeyboardWillHideNotification, object: nil)
}
private var internalScrollView: UIScrollView! {
get {
return objc_getAssociatedObject(self, &scrollViewKey) as? UIScrollView
}
set(newValue) {
objc_setAssociatedObject(self, &scrollViewKey, newValue, UInt(OBJC_ASSOCIATION_ASSIGN))
}
}
func keyboardWillShow(notification: NSNotification) {
let userInfo = notification.userInfo as! Dictionary<String, AnyObject>
let animationDuration = userInfo[UIKeyboardAnimationDurationUserInfoKey] as! NSTimeInterval
let animationCurve = userInfo[UIKeyboardAnimationCurveUserInfoKey]!.intValue
let keyboardFrame = userInfo[UIKeyboardFrameEndUserInfoKey]?.CGRectValue()
let keyboardFrameConvertedToViewFrame = view.convertRect(keyboardFrame!, fromView: nil)
let curveAnimationOption = UIViewAnimationOptions(UInt(animationCurve))
let options = UIViewAnimationOptions.BeginFromCurrentState | curveAnimationOption
UIView.animateWithDuration(animationDuration, delay: 0, options:options, animations: { () -> Void in
let insetHeight = (self.internalScrollView.frame.height + self.internalScrollView.frame.origin.y) - keyboardFrameConvertedToViewFrame.origin.y
self.internalScrollView.contentInset = UIEdgeInsetsMake(0, 0, insetHeight, 0)
self.internalScrollView.scrollIndicatorInsets = UIEdgeInsetsMake(0, 0, insetHeight, 0)
}) { (complete) -> Void in
}
}
func keyboardWillHide(notification: NSNotification) {
let userInfo = notification.userInfo as! Dictionary<String, AnyObject>
let animationDuration = userInfo[UIKeyboardAnimationDurationUserInfoKey] as! NSTimeInterval
let animationCurve = userInfo[UIKeyboardAnimationCurveUserInfoKey]!.intValue
let keyboardFrame = userInfo[UIKeyboardFrameEndUserInfoKey]?.CGRectValue()
let keyboardFrameConvertedToViewFrame = view.convertRect(keyboardFrame!, fromView: nil)
let curveAnimationOption = UIViewAnimationOptions(UInt(animationCurve))
let options = UIViewAnimationOptions.BeginFromCurrentState | curveAnimationOption
UIView.animateWithDuration(animationDuration, delay: 0, options:options, animations: { () -> Void in
self.internalScrollView.contentInset = UIEdgeInsetsMake(0, 0, 0, 0)
self.internalScrollView.scrollIndicatorInsets = UIEdgeInsetsMake(0, 0, 0, 0)
}) { (complete) -> Void in
}
}
}
| apache-2.0 | e0672c5cd4d92be917d69aceab205fb3 | 53.546875 | 154 | 0.733314 | 5.847571 | false | false | false | false |
CesarValiente/CursoSwiftUniMonterrey | week3/week3_enums.playground/Contents.swift | 1 | 782 | //: Week3 -- Enums
import UIKit
//let cities = ["Cancun", "Guadalajara", "DF", "Monterrey"]
//
//enum City {
// case Cancun, Guadalajara, DF, Monterrey
//}
//
//func getCiudad (ciudad : City) -> String {
// switch ciudad {
// case .Cancun:
// return "Beach city"
// case .Guadalajara, .DF, .Monterrey:
// return "Non beach city"
// default:
// return "Non valid option"
// }
//}
//
//getCiudad(City.Cancun)
let cities = ["Cancun", "Guadalajara", "DF", "Monterrey"]
enum City : Int {
case Cancun = 450, Guadalajara = 10, DF = 8, Monterrey = 500, Merida
}
func calculateDistance (city : City) -> Int {
return City.Cancun.rawValue - city.rawValue
}
calculateDistance(City.Merida)
City.Merida.rawValue
| mit | 89f15ed23d83e8f7c9672e23215ad1a5 | 19.578947 | 72 | 0.590793 | 3.115538 | false | false | false | false |
cemolcay/ReorderableGridView-Swift | ReorderableGridView-Swift/MultipleGridViewController.swift | 1 | 6312 | //
// MultipleGridViewController.swift
// ReorderableGridView-Swift
//
// Created by Cem Olcay on 21/11/14.
// Copyright (c) 2014 Cem Olcay. All rights reserved.
//
import UIKit
class MultipleGridViewController: UIViewController, Draggable {
// MARK: Properties
@IBOutlet var selectedItemsGridContainerView: UIView!
@IBOutlet var itemsGridContainerView: UIView!
var selectedItemsGrid: ReorderableGridView?
var itemsGrid: ReorderableGridView?
var itemCount: Int = 0
var borderColor: UIColor?
var bgColor: UIColor?
var bottomColor: UIColor?
// MARK: Lifecycle
override func viewDidLoad() {
super.viewDidLoad()
borderColor = RGBColor(233, g: 233, b: 233)
bgColor = RGBColor(242, g: 242, b: 242)
bottomColor = RGBColor(65, g: 65, b: 65)
self.title = "Multiple Grid"
self.navigationItem.title = "Multiple Grid View Demo"
self.view.backgroundColor = bgColor
selectedItemsGrid = ReorderableGridView (frame: selectedItemsGridContainerView.bounds, itemWidth: 180)
selectedItemsGrid?.draggableDelegate = self
selectedItemsGrid?.reorderable = false
selectedItemsGrid?.clipsToBounds = false
selectedItemsGridContainerView.addSubview(selectedItemsGrid!)
itemsGrid = ReorderableGridView (frame: itemsGridContainerView.bounds, itemWidth: 180)
itemsGrid?.draggableDelegate = self
itemsGrid?.clipsToBounds = false
itemsGrid?.reorderable = false
itemsGridContainerView.addSubview(itemsGrid!)
for _ in 0..<20 {
itemsGrid?.addReorderableView(itemView())
}
}
override func viewDidAppear(animated: Bool) {
super.viewDidAppear(animated)
if let grid = itemsGrid {
grid.frame = itemsGridContainerView.bounds
grid.invalidateLayout()
}
if let grid = selectedItemsGrid {
grid.frame = selectedItemsGridContainerView.bounds
grid.invalidateLayout()
}
}
func itemView () -> ReorderableView {
let w : CGFloat = 180
let h : CGFloat = 100 + CGFloat(arc4random()%100)
let view = ReorderableView (x: 0, y: 0, w: w, h: h)
view.tag = itemCount++
view.layer.borderColor = borderColor?.CGColor
view.layer.backgroundColor = UIColor.whiteColor().CGColor
view.layer.borderWidth = 1
view.layer.cornerRadius = 5
view.layer.masksToBounds = true
let topView = UIView(x: 0, y: 0, w: view.w, h: 50)
view.addSubview(topView)
let itemLabel = UILabel (frame: topView.frame)
itemLabel.center = topView.center
itemLabel.font = UIFont.HelveticaNeue(.Thin, size: 20)
itemLabel.textAlignment = NSTextAlignment.Center
itemLabel.textColor = bottomColor?
itemLabel.text = "\(view.tag)"
itemLabel.layer.masksToBounds = true
topView.addSubview(itemLabel)
let sepLayer = CALayer ()
sepLayer.frame = CGRect (x: 0, y: topView.bottom, width: topView.w, height: 1)
sepLayer.backgroundColor = borderColor?.CGColor
topView.layer.addSublayer(sepLayer)
let bottomView = UIView(frame: CGRect(x: 0, y: topView.bottom, width: view.w, height: view.h-topView.h))
let bottomLayer = CALayer ()
bottomLayer.frame = CGRect (x: 0, y: bottomView.h-5, width: bottomView.w, height: 5)
bottomLayer.backgroundColor = bottomColor?.CGColor
bottomView.layer.addSublayer(bottomLayer)
bottomView.layer.masksToBounds = true
view.addSubview(bottomView)
return view
}
// MARK: Draggable
func didDragStartedForView(reorderableGridView: ReorderableGridView, view: ReorderableView) {
}
func didDraggedView(reorderableGridView: ReorderableGridView, view: ReorderableView) {
}
func didDragEndForView(reorderableGridView: ReorderableGridView, view: ReorderableView) {
// items grid to selected items grid
if (reorderableGridView == itemsGrid!) {
let convertedPos : CGPoint = self.view.convertPoint(view.center, fromView: itemsGridContainerView)
if (CGRectContainsPoint(selectedItemsGridContainerView.frame, convertedPos)) {
itemsGrid?.removeReorderableView(view)
selectedItemsGrid?.addReorderableView(view)
} else {
reorderableGridView.invalidateLayout()
}
}
// selected items grid to items grid
else if (reorderableGridView == selectedItemsGrid!) {
let convertedPos : CGPoint = self.view.convertPoint(view.center, fromView: selectedItemsGridContainerView)
if (CGRectContainsPoint(itemsGridContainerView.frame, convertedPos)) {
selectedItemsGrid?.removeReorderableView(view)
itemsGrid?.addReorderableView(view)
} else {
reorderableGridView.invalidateLayout()
}
}
}
// MARK: Interface Rotation
override func didRotateFromInterfaceOrientation(fromInterfaceOrientation: UIInterfaceOrientation) {
selectedItemsGrid?.frame = selectedItemsGridContainerView.bounds
itemsGrid?.frame = itemsGridContainerView.bounds
selectedItemsGrid?.invalidateLayout()
itemsGrid?.invalidateLayout()
}
// MARK: Utils
func randomColor () -> UIColor {
var randomRed:CGFloat = CGFloat(drand48())
var randomGreen:CGFloat = CGFloat(drand48())
var randomBlue:CGFloat = CGFloat(drand48())
return UIColor(red: randomRed, green: randomGreen, blue: randomBlue, alpha: 1.0)
}
func RGBColor(r: CGFloat, g: CGFloat, b: CGFloat) -> UIColor {
return UIColor (red: r / 255.0, green: g / 255.0, blue: b / 255.0, alpha: 1)
}
func RGBAColor (r: CGFloat, g: CGFloat, b: CGFloat, a: CGFloat) -> UIColor {
return UIColor (red: r / 255.0, green: g / 255.0, blue: b / 255.0, alpha: a)
}
}
| mit | 1262375aba21c04dbebc9bd59b4182ee | 33.681319 | 118 | 0.627535 | 5.273183 | false | false | false | false |
swift-gtk/SwiftGTK | Sources/UIKit/GList.swift | 1 | 315 |
extension Array {
internal init(gList: UnsafeMutablePointer<GList>?) {
var list = gList
var data = [Element]()
if list?.pointee.data != nil {
repeat {
data.append(unsafeBitCast(list!.pointee.data, to: Element.self))
list = list!.pointee.next
} while list != nil
}
self.init(data)
}
}
| gpl-2.0 | 38afc8e7faaf70e90fad3875d12e1edf | 16.5 | 68 | 0.64127 | 3.088235 | false | false | false | false |
nanthi1990/SwiftCharts | SwiftCharts/Axis/ChartAxisXLowLayerDefault.swift | 7 | 1104 | //
// ChartAxisXLowLayerDefault.swift
// SwiftCharts
//
// Created by ischuetz on 25/04/15.
// Copyright (c) 2015 ivanschuetz. All rights reserved.
//
import UIKit
class ChartAxisXLowLayerDefault: ChartAxisXLayerDefault {
override var low: Bool {return true}
override var lineP1: CGPoint {
return self.p1
}
override var lineP2: CGPoint {
return self.p2
}
override func chartViewDrawing(#context: CGContextRef, chart: Chart) {
super.chartViewDrawing(context: context, chart: chart)
}
override func initDrawers() {
self.lineDrawer = self.generateLineDrawer(offset: 0)
let labelsOffset = (self.settings.axisStrokeWidth / 2) + self.settings.labelsToAxisSpacingX
let labelDrawers = self.generateLabelDrawers(offset: labelsOffset)
let definitionLabelsOffset = labelsOffset + self.labelsTotalHeight + self.settings.axisTitleLabelsToLabelsSpacing
self.axisTitleLabelDrawers = self.generateAxisTitleLabelsDrawers(offset: definitionLabelsOffset)
self.labelDrawers = labelDrawers
}
}
| apache-2.0 | 9b9816724e56164268339989332a7666 | 30.542857 | 121 | 0.711957 | 4.697872 | false | false | false | false |
SiddharthChopra/KahunaSocialMedia | KahunaSocialMedia/Classes/Twitter/TwitterDataInfo.swift | 1 | 426 | //
// TwitterDataInfo.swift
// MyCity311
//
// Created by Piyush on 6/15/16.
// Copyright © 2016 Kahuna Systems. All rights reserved.
//
import UIKit
public class TwitterDataInfo: NSObject {
public var profileIcon = String()
public var tweetDate = String()
public var tweeterUserId = String()
public var tweeterUserName = String()
public var tweetId: String?
public var tweetText = String()
}
| mit | 839aad4d485ab8b39b4d4ce7aaae3e56 | 20.25 | 57 | 0.689412 | 3.794643 | false | false | false | false |
xwu/swift | stdlib/public/Concurrency/AsyncThrowingDropWhileSequence.swift | 1 | 5613 | //===----------------------------------------------------------------------===//
//
// This source file is part of the Swift.org open source project
//
// Copyright (c) 2021 Apple Inc. and the Swift project authors
// Licensed under Apache License v2.0 with Runtime Library Exception
//
// See https://swift.org/LICENSE.txt for license information
// See https://swift.org/CONTRIBUTORS.txt for the list of Swift project authors
//
//===----------------------------------------------------------------------===//
import Swift
@available(SwiftStdlib 5.5, *)
extension AsyncSequence {
/// Omits elements from the base sequence until a given error-throwing closure
/// returns false, after which it passes through all remaining elements.
///
/// Use `drop(while:)` to omit elements from an asynchronous sequence until
/// the element received meets a condition you specify. If the closure you
/// provide throws an error, the sequence produces no elements and throws
/// the error instead.
///
/// In this example, an asynchronous sequence called `Counter` produces `Int`
/// values from `1` to `10`. The predicate passed to the `drop(while:)`
/// method throws an error if it encounters an even number, and otherwise
/// returns `true` while it receives elements less than `5`. Because the
/// predicate throws when it receives `2` from the base sequence, this example
/// throws without ever printing anything.
///
/// do {
/// let stream = Counter(howHigh: 10)
/// .drop {
/// if $0 % 2 == 0 {
/// throw EvenError()
/// }
/// return $0 < 5
/// }
/// for try await number in stream {
/// print("\(number) ")
/// }
/// } catch {
/// print ("\(error)")
/// }
/// // Prints: EvenError()
///
/// After the predicate returns `false`, the sequence never executes it again,
/// and from then on the sequence passes through elements from its underlying
/// sequence. A predicate that throws an error also never executes again.
///
/// - Parameter predicate: An error-throwing closure that takes an element as
/// a parameter and returns a Boolean value indicating whether to drop the
/// element from the modified sequence.
/// - Returns: An asynchronous sequence that skips over values until the
/// provided closure returns `false` or throws an error.
@inlinable
public __consuming func drop(
while predicate: @escaping (Element) async throws -> Bool
) -> AsyncThrowingDropWhileSequence<Self> {
AsyncThrowingDropWhileSequence(self, predicate: predicate)
}
}
/// An asynchronous sequence which omits elements from the base sequence until a
/// given error-throwing closure returns false, after which it passes through
/// all remaining elements.
@available(SwiftStdlib 5.5, *)
public struct AsyncThrowingDropWhileSequence<Base: AsyncSequence> {
@usableFromInline
let base: Base
@usableFromInline
let predicate: (Base.Element) async throws -> Bool
@usableFromInline
init(
_ base: Base,
predicate: @escaping (Base.Element) async throws -> Bool
) {
self.base = base
self.predicate = predicate
}
}
@available(SwiftStdlib 5.5, *)
extension AsyncThrowingDropWhileSequence: AsyncSequence {
/// The type of element produced by this asynchronous sequence.
///
/// The drop-while sequence produces whatever type of element its base
/// sequence produces.
public typealias Element = Base.Element
/// The type of iterator that produces elements of the sequence.
public typealias AsyncIterator = Iterator
/// The iterator that produces elements of the drop-while sequence.
public struct Iterator: AsyncIteratorProtocol {
@usableFromInline
var baseIterator: Base.AsyncIterator
@usableFromInline
let predicate: (Base.Element) async throws -> Bool
@usableFromInline
var finished = false
@usableFromInline
var doneDropping = false
@usableFromInline
init(
_ baseIterator: Base.AsyncIterator,
predicate: @escaping (Base.Element) async throws -> Bool
) {
self.baseIterator = baseIterator
self.predicate = predicate
}
/// Produces the next element in the drop-while sequence.
///
/// This iterator calls `next()` on its base iterator and evaluates the
/// result with the `predicate` closure. As long as the predicate returns
/// `true`, this method returns `nil`. After the predicate returns `false`,
/// for a value received from the base iterator, this method returns that
/// value. After that, the iterator returns values received from its
/// base iterator as-is, and never executes the predicate closure again.
/// If calling the closure throws an error, the sequence ends and `next()`
/// rethrows the error.
@inlinable
public mutating func next() async throws -> Base.Element? {
while !finished && !doneDropping {
guard let element = try await baseIterator.next() else {
return nil
}
do {
if try await predicate(element) == false {
doneDropping = true
return element
}
} catch {
finished = true
throw error
}
}
guard !finished else {
return nil
}
return try await baseIterator.next()
}
}
@inlinable
public __consuming func makeAsyncIterator() -> Iterator {
return Iterator(base.makeAsyncIterator(), predicate: predicate)
}
}
| apache-2.0 | 68dc9b3e5a13e48e71d6befe2a5cec91 | 34.980769 | 80 | 0.642081 | 4.919369 | false | false | false | false |
yongju-hong/thrift | lib/swift/Sources/TStruct.swift | 11 | 3466 | /*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
/// Protocol for Generated Structs to conform to
/// Dictionary maps field names to internal IDs and uses Reflection
/// to iterate through all fields.
/// `writeFieldValue(_:name:type:id:)` calls `TSerializable.write(to:)` internally
/// giving a nice recursive behavior for nested TStructs, TLists, TMaps, and TSets
public protocol TStruct : TSerializable {
static var fieldIds: [String: Int32] { get }
static var structName: String { get }
}
public extension TStruct {
static var fieldIds: [String: (id: Int32, type: TType)] { return [:] }
static var thriftType: TType { return .struct }
func write(to proto: TProtocol) throws {
// Write struct name first
try proto.writeStructBegin(name: Self.structName)
try self.forEach { name, value, id in
// Write to protocol
try proto.writeFieldValue(value, name: name,
type: value.thriftType, id: id)
}
try proto.writeFieldStop()
try proto.writeStructEnd()
}
/// Provides a block for handling each (available) thrift property using reflection
/// Caveat: Skips over optional values
/// Provides a block for handling each (available) thrift property using reflection
///
/// - parameter block: block for handling property
///
/// - throws: rethrows any Error thrown in block
private func forEach(_ block: (_ name: String, _ value: TSerializable, _ id: Int32) throws -> Void) rethrows {
// Mirror the object, getting (name: String?, value: Any) for every property
let mirror = Mirror(reflecting: self)
// Iterate through all children, ignore empty property names
for (propName, propValue) in mirror.children {
guard let propName = propName else { continue }
if let tval = unwrap(any: propValue, parent: mirror) as? TSerializable, let id = Self.fieldIds[propName] {
try block(propName, tval, id)
}
}
}
/// Any can mysteriously be an Optional<Any> at the same time,
/// this checks and always returns Optional<Any> without double wrapping
/// we then try to bind value as TSerializable to ignore any extension properties
/// and the like and verify the property exists and grab the Thrift
/// property ID at the same time
///
/// - parameter any: Any instance to attempt to unwrap
///
/// - returns: Unwrapped Any as Optional<Any>
private func unwrap(any: Any, parent: Mirror) -> Any? {
let mi = Mirror(reflecting: any)
if parent.displayStyle != .enum && mi.displayStyle != .optional { return any }
if mi.children.count == 0 { return nil }
let (_, some) = mi.children.first!
return some
}
}
| apache-2.0 | d26755d0fea7dcee7d23b12e5da2cb77 | 37.087912 | 112 | 0.690421 | 4.348808 | false | false | false | false |
LYM-mg/MGDS_Swift | MGDS_Swift/MGDS_Swift/Class/Home/More/View/MoreContentView.swift | 1 | 6483 | //
// MoreContentView.swift
// MGDS_Swift
//
// Created by i-Techsys.com on 2017/7/5.
// Copyright © 2017年 i-Techsys. All rights reserved.
//
import UIKit
@objc
protocol MoreContentViewDelegate : class {
func MoreContentViewDidScroll(contentView : MoreContentView, progress : CGFloat, sourceIndex : Int, targetIndex : Int)
@objc optional func contentViewEndScroll(_ contentView : MoreContentView)
}
private let ContentCellID = "ContentCellID"
class MoreContentView: UIView {
// MARK:- 定义属性
fileprivate var childVcs : [UIViewController]
weak var parentViewController : UIViewController? /** 当前显示的父控制器 */
fileprivate var startOffsetX : CGFloat = 0
fileprivate var isForbidScrollDelegate : Bool = false
weak var delegate : MoreContentViewDelegate?
// MARK:- 懒加载属性
fileprivate lazy var collectionView : UICollectionView = {[weak self] in
// 1.创建layout
let layout = UICollectionViewFlowLayout()
layout.itemSize = (self?.bounds.size)!
layout.minimumLineSpacing = 0
layout.minimumInteritemSpacing = 0
layout.scrollDirection = .horizontal
// 2.创建UICollectionView
let collectionView = UICollectionView(frame: CGRect.zero, collectionViewLayout: layout)
collectionView.showsHorizontalScrollIndicator = false
collectionView.isPagingEnabled = true
collectionView.bounces = false
collectionView.scrollsToTop = false
collectionView.dataSource = self
collectionView.delegate = self
collectionView.register(UICollectionViewCell.classForCoder(), forCellWithReuseIdentifier: ContentCellID)
return collectionView
}()
// MARK:- 自定义构造函数
init(frame: CGRect, childVcs: [UIViewController], parentViewController : UIViewController?) {
self.childVcs = childVcs
self.parentViewController = parentViewController
super.init(frame: frame)
self.backgroundColor = UIColor.clear
setUpUI()
}
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
}
// MARK:- 初始化UI
extension MoreContentView {
fileprivate func setUpUI() {
// 1.将所有的子控制器添加父控制器中
for childVc in childVcs {
parentViewController?.addChild(childVc)
}
// 2.添加UICollectionView,用于在Cell中存放控制器的View
addSubview(collectionView)
collectionView.frame = bounds
}
}
// MARK:- UICollectionViewDataSource
extension MoreContentView: UICollectionViewDataSource {
func numberOfSections(in collectionView: UICollectionView) -> Int {
return 1
}
func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int {
return childVcs.count
}
func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
// 1.创建Cell
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: ContentCellID, for: indexPath) as UICollectionViewCell
// 2.给Cell设置内容
for view in cell.contentView.subviews {
view.removeFromSuperview()
}
let childVc = childVcs[(indexPath as NSIndexPath).item]
childVc.view.frame = cell.contentView.bounds
childVc.view.backgroundColor = UIColor.white
cell.contentView.addSubview(childVc.view)
return cell
}
}
// MARK:- 遵守UICollectionViewDelegate
extension MoreContentView : UICollectionViewDelegate {
func scrollViewWillBeginDragging(_ scrollView: UIScrollView) {
isForbidScrollDelegate = false
startOffsetX = scrollView.contentOffset.x
}
func scrollViewDidScroll(_ scrollView: UIScrollView) {
// 0.判断是否是点击事件
if isForbidScrollDelegate { return }
// 1.定义获取需要的数据
var progress : CGFloat = 0
var sourceIndex : Int = 0
var targetIndex : Int = 0
// 2.判断是左滑还是右滑
let currentOffsetX = scrollView.contentOffset.x
let scrollViewW = scrollView.bounds.width
if currentOffsetX > startOffsetX { // 左滑
// 1.计算progress
progress = currentOffsetX / scrollViewW - floor(currentOffsetX / scrollViewW)
// 2.计算sourceIndex
sourceIndex = Int(currentOffsetX / scrollViewW)
// 3.计算targetIndex
targetIndex = sourceIndex + 1
if targetIndex >= childVcs.count {
targetIndex = childVcs.count - 1
}
// 4.如果完全划过去
if currentOffsetX - startOffsetX == scrollViewW {
progress = 1
targetIndex = sourceIndex
}
} else { // 右滑
// 1.计算progress
progress = 1 - (currentOffsetX / scrollViewW - floor(currentOffsetX / scrollViewW))
// 2.计算targetIndex
targetIndex = Int(currentOffsetX / scrollViewW)
// 3.计算sourceIndex
sourceIndex = targetIndex + 1
if sourceIndex >= childVcs.count {
sourceIndex = childVcs.count - 1
}
}
// 3.将progress/sourceIndex/targetIndex传递给titleView
delegate?.MoreContentViewDidScroll(contentView: self, progress: progress, sourceIndex: sourceIndex, targetIndex: targetIndex)
}
func scrollViewDidEndDecelerating(_ scrollView: UIScrollView) {
delegate?.contentViewEndScroll?(self)
}
func scrollViewDidEndDragging(_ scrollView: UIScrollView, willDecelerate decelerate: Bool) {
if !decelerate {
delegate?.contentViewEndScroll?(self)
}
}
}
// MARK:- 对外暴露的方法
extension MoreContentView {
func setCurrentIndex(currentIndex : Int) {
// 1.记录需要进制执行代理方法
isForbidScrollDelegate = true
// 2.滚动正确的位置
let offsetX = CGFloat(currentIndex) * collectionView.frame.width
collectionView.setContentOffset(CGPoint(x: offsetX, y: 0), animated: false)
}
}
| mit | 77bc89da0613dfea1f574b1e6e9e80cf | 32.069519 | 133 | 0.638422 | 5.85052 | false | false | false | false |
JJCoderiOS/DDMusic | DDMusic/Tools/MusicOperationTool.swift | 1 | 4321 |
import UIKit
import MediaPlayer
/* 音乐操作工具 */
class MusicOperationTool: NSObject {
//单例 数据共享
static let shareInstance = MusicOperationTool()
//工具类
let tool : MusicTool = MusicTool()
var artWork : MPMediaItemArtwork?
/* 上次歌词行号,防止多次加载绘制歌词到图片上 */
var lastLrcRow = -1
var musicModel : MusicMessageModel = MusicMessageModel()
//锁屏时候获取的信息
func getMusicMessage() ->MusicMessageModel {
musicModel.modelM = musicsM[currentPlayMusicIndex]
musicModel.costTime = (tool.player?.currentTime) ?? 0
musicModel.totalTime = (tool.player?.duration) ?? 0
musicModel.isPlaying = (tool.player?.isPlaying) ?? false
return musicModel
}
/* 列表数组 */
var musicsM : [MusicModel] = [MusicModel]()
var currentPlayMusicIndex = -1 {
didSet {
if currentPlayMusicIndex < 0 {
currentPlayMusicIndex = musicsM.count - 1
}
if currentPlayMusicIndex > musicsM.count - 1 {
currentPlayMusicIndex = 0
}
}
}
/* 播放音乐 */
func playMusic(musics : MusicModel) {
tool.playMusicWithName(musicName: musics.fileName!)
currentPlayMusicIndex = musicsM.index(of: musics)!
print("当前的播放的是第几首歌曲", (currentPlayMusicIndex))
}
/* 详情界面调用 播放当前音乐 */
func playCurrnetMusic () {
let model = musicsM[currentPlayMusicIndex]
playMusic(musics: model)
}
/* 暂停音乐 */
func pauseCurrnentMusic() {
tool.pauseMusic()
}
/* 下一首音乐 */
func nextMusic() {
/* 下一个数据 */
currentPlayMusicIndex += 1
//取出数据
let model = musicsM[currentPlayMusicIndex]
playMusic(musics: model)
}
/* 上一首音乐 */
func preMusic() {
/* 上一个数据 */
currentPlayMusicIndex -= 1
//取出数据
let model = musicsM[currentPlayMusicIndex]
playMusic(musics: model)
}
/* 设置播放比例是多少 界面滑块滑动或者点击时候触发 */
func setPlayerPlayRotate(progress : CGFloat) {
if tool.getCurrnetPlayerPlayRotate() == progress {
return
}
let duration = tool.player?.duration
tool.player?.currentTime = TimeInterval(progress) * duration!
}
}
/* 锁屏解密通信 */
extension MusicOperationTool {
func setUpLocakMessage() -> () {
/* 可以不将歌词添加到图片 */
let socket = true
let message = getMusicMessage()
let mediaCenter = MPNowPlayingInfoCenter.default()
//标题
let title = message.modelM?.name ?? ""
let singerName = message.modelM?.singer ?? ""
let costTime = message.costTime
let totalTime = message.totalTime
let iconName = message.modelM?.singerIcon ?? ""
//获取歌词
let lrcName = message.modelM?.lrcName
let lrcMs = MusicDataTool.getLrcMS(lrcName: lrcName)
let lrcM = MusicDataTool.getCurrntLrcModel(currentTime: message.costTime, lrcModel: lrcMs).model
let lrcRow = MusicDataTool.getCurrntLrcModel(currentTime: message.costTime, lrcModel: lrcMs).row
var image = UIImage(named: iconName)
var dic : [String : Any] = [
MPMediaItemPropertyTitle : title,
MPMediaItemPropertyArtist : singerName,
MPMediaItemPropertyPlaybackDuration : totalTime,
MPNowPlayingInfoPropertyElapsedPlaybackTime : costTime,
]
if socket && lrcRow != lastLrcRow && image != nil {
lastLrcRow = lrcRow
image = LrcAddImageTool.addLrcToImage(lrc: lrcM?.conent, sourceImage: image)
if image != nil {
artWork = MPMediaItemArtwork(image: image!)
}
}
if artWork != nil {
dic[MPMediaItemPropertyArtwork] = artWork
}
mediaCenter.nowPlayingInfo = dic
//开始接受远程事件
UIApplication.shared.beginReceivingRemoteControlEvents()
}
}
| apache-2.0 | de44fafa55391139eb46c5f30c7769ca | 29.496183 | 104 | 0.590238 | 4.623843 | false | false | false | false |
diwu/LeetCode-Solutions-in-Swift | Solutions/SolutionsTests/Easy/Easy_088_Merge_Sorted_Array_Test.swift | 1 | 1993 | //
// Easy_088_Merge_Sorted_Array_Test.swift
// Solutions
//
// Created by Di Wu on 10/15/15.
// Copyright © 2015 diwu. All rights reserved.
//
import XCTest
class Easy_088_Merge_Sorted_Array_Test: XCTestCase, SolutionsTestCase {
func test_001() {
var input0: [Int] = [0]
let input1: [Int] = [1]
let m: Int = 0
let n: Int = 1
let expected: [Int] = [1]
asyncHelper(input0: &input0, input1: input1, m: m, n: n, expected: expected)
}
func test_002() {
var input0: [Int] = [1]
let input1: [Int] = [0]
let m: Int = 1
let n: Int = 0
let expected: [Int] = [1]
asyncHelper(input0: &input0, input1: input1, m: m, n: n, expected: expected)
}
func test_003() {
var input0: [Int] = [1, 3, 5, 7, 9, 0, 0, 0, 0, 0]
let input1: [Int] = [2, 4, 6, 8, 10]
let m: Int = 5
let n: Int = 5
let expected: [Int] = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
asyncHelper(input0: &input0, input1: input1, m: m, n: n, expected: expected)
}
private func asyncHelper(input0: inout [Int], input1: [Int], m: Int, n: Int, expected: [Int]) {
weak var expectation: XCTestExpectation? = self.expectation(description:timeOutName())
var localInput0 = input0
serialQueue().async(execute: { () -> Void in
Easy_088_Merge_Sorted_Array.merge(nums1: &localInput0, m: m, nums2: input1, n: n)
assertHelper(localInput0 == expected, problemName:self.problemName(), input: localInput0, resultValue: localInput0, expectedValue: expected)
if let unwrapped = expectation {
unwrapped.fulfill()
}
})
waitForExpectations(timeout:timeOut()) { (error: Error?) -> Void in
if error != nil {
assertHelper(false, problemName:self.problemName(), input: localInput0, resultValue:self.timeOutName(), expectedValue: expected)
}
}
}
}
| mit | 7837d3095fe5e25aa4a0fc1ca24c5c0c | 37.307692 | 152 | 0.565261 | 3.382003 | false | true | false | false |
jovito-royeca/CardMagusKit | CardMagusKit/Classes/NetworkingManager.swift | 1 | 8231 | //
// NetworkingManager.swift
// Flag Ceremony
//
// Created by Jovit Royeca on 01/11/2016.
// Copyright © 2016 Jovit Royeca. All rights reserved.
//
import UIKit
import Networking
import ReachabilitySwift
enum HTTPMethod: String {
case post = "Post",
get = "Get",
head = "Head",
put = "Put"
}
typealias NetworkingResult = (_ result: [[String : Any]], _ error: NSError?) -> Void
class NetworkingManager: NSObject {
// var networkers = [String: Any]()
let reachability = Reachability()!
func doOperation(_ baseUrl: String,
path: String,
method: HTTPMethod,
headers: [String: String]?,
paramType: Networking.ParameterType,
params: AnyObject?,
completionHandler: @escaping NetworkingResult) -> Void {
if !reachability.isReachable {
let error = NSError(domain: "network", code: 408, userInfo: [NSLocalizedDescriptionKey: "Network error."])
completionHandler([[String : Any]](), error)
} else {
let networker = networking(forBaseUrl: baseUrl)
if let headers = headers {
networker.headerFields = headers
}
switch (method) {
case .post:
networker.post(path, parameterType: paramType, parameters: params, completion: {(result) in
switch result {
case .success(let response):
if response.json.dictionary.count > 0 {
completionHandler([response.json.dictionary], nil)
} else if response.json.array.count > 0 {
completionHandler(response.json.array, nil)
} else {
completionHandler([[String : Any]](), nil)
}
case .failure(let response):
let error = response.error
print("Networking error: \(error)")
completionHandler([[String : Any]](), error)
}
})
case .get:
networker.get(path, parameters: params, completion: {(result) in
switch result {
case .success(let response):
if response.json.dictionary.count > 0 {
completionHandler([response.json.dictionary], nil)
} else if response.json.array.count > 0 {
completionHandler(response.json.array, nil)
} else {
completionHandler([[String : Any]](), nil)
}
case .failure(let response):
let error = response.error
print("Networking error: \(error)")
completionHandler([[String : Any]](), error)
}
})
case .head:
()
case .put:
networker.put(path, parameterType: paramType, parameters: params, completion: {(result) in
switch result {
case .success(let response):
if response.json.dictionary.count > 0 {
completionHandler([response.json.dictionary], nil)
} else if response.json.array.count > 0 {
completionHandler(response.json.array, nil)
} else {
completionHandler([[String : Any]](), nil)
}
case .failure(let response):
let error = response.error
print("Networking error: \(error)")
completionHandler([[String : Any]](), error)
}
})
}
}
}
func downloadImage(_ url: URL, completionHandler: @escaping (_ origURL: URL?, _ image: UIImage?, _ error: NSError?) -> Void) {
let networker = networking(forUrl: url)
var path = url.path
if let query = url.query {
path += "?\(query)"
}
networker.downloadImage(path, completion: {(result) in
switch result {
case .success(let response):
// skip from iCloud backups!
do {
var destinationURL = try networker.destinationURL(for: path)
var resourceValues = URLResourceValues()
resourceValues.isExcludedFromBackup = true
try destinationURL.setResourceValues(resourceValues)
}
catch {}
completionHandler(url, response.image, nil)
case .failure(let response):
let error = response.error
print("Networking error: \(error)")
completionHandler(nil, nil, error)
}
})
}
func localImageFromURL(_ url: URL) -> UIImage? {
let networker = networking(forUrl: url)
var path = url.path
if let query = url.query {
path += "?\(query)"
}
do {
let destinationURL = try networker.destinationURL(for: path)
if FileManager.default.fileExists(atPath: destinationURL.path) {
return UIImage(contentsOfFile: destinationURL.path)
}
} catch {}
return nil
}
func downloadFile(_ url: URL, completionHandler: @escaping (Data?, NSError?) -> Void) {
let networker = networking(forUrl: url)
let path = url.path
networker.downloadData(path, cacheName: nil, completion: {(result) in
switch result {
case .success(let response):
completionHandler(response.data, nil)
case .failure(let response):
let error = response.error
print("Networking error: \(error)")
completionHandler(nil, error)
}
})
}
func fileExistsAt(_ url : URL, completion: @escaping (Bool) -> Void) {
let checkSession = Foundation.URLSession.shared
var request = URLRequest(url: url)
request.httpMethod = "HEAD"
request.timeoutInterval = 1.0 // Adjust to your needs
let task = checkSession.dataTask(with: request as URLRequest, completionHandler: { (data, response, error) -> Void in
if let httpResp: HTTPURLResponse = response as? HTTPURLResponse {
completion(httpResp.statusCode == 200)
}
})
task.resume()
}
// MARK: Private methods
fileprivate func networking(forBaseUrl url: String) -> Networking {
var networker:Networking?
//
// if let n = networkers[url] as? Networking {
// networker = n
// } else {
// let newN = Networking(baseURL: url, configurationType: .default)
//
// networkers[url] = newN
// networker = newN
// }
//
networker = Networking(baseURL: url, configurationType: .default)
return networker!
}
fileprivate func networking(forUrl url: URL) -> Networking {
var networker:Networking?
var baseUrl = ""
if let scheme = url.scheme {
baseUrl = "\(scheme)://"
}
if let host = url.host {
baseUrl.append(host)
}
// if let n = networkers[baseUrl] as? Networking {
// networker = n
// } else {
// let newN = Networking(baseURL: baseUrl, configurationType: .default)
//
// networkers[baseUrl] = newN
// networker = newN
// }
networker = Networking(baseURL: baseUrl, configurationType: .default)
return networker!
}
// MARK: - Shared Instance
static let sharedInstance = NetworkingManager()
}
| mit | 52294bd6742ed29bf1eedf525d496f62 | 36.072072 | 130 | 0.49842 | 5.516086 | false | false | false | false |
icapps/ios_objective_c_workshop | Teacher/ObjcTextInput/Pods/Faro/Sources/Mocking/RequestStub.swift | 2 | 4231 | //
// RequestStub.swift
// Horizon Go
//
// Created by Jelle Vandebeeck on 14/06/2017.
// Copyright © 2017 Jelle Vandebeeck. All rights reserved.
//
import Foundation
/// This reponse contains the stubbed data and status code that is returned for a given path.
public struct StubbedResponse {
// The response's data.
var data: Data?
// The response's status code
var statusCode: Int
}
/// The `RequestStub` handles everything that has to do with stubbing the requests, from swizzling the
/// `URLSessionConfiguration` to registering the different stubs.
public class RequestStub {
// MARK: - Internals
private var responses = [String: [StubbedResponse]]()
// MARK: - Init
/// You should always use this singleton method to register stubs. This is needed because we need to be able to
/// fetch the `responses` from within the `StubbedURLProtocol` class.
public static var shared = RequestStub()
public init() {
}
// MARK: - Class
public class func removeAllStubs() {
shared.removeAllStubs()
}
// MARK: - Mutating
/// Append a body with status code to a given path. When calling this method multiple times for the same path
/// these stubbed requests will be triggered sequentially.
///
/// - Parameters:
/// - path: The path of the request, should be prefixid with "/"
/// - statusCode: The stubbed status code
/// - body: The stubbed JSON body code
internal func append(path: String, statusCode: Int, dictionary: [String: Any]?) {
var data: Data?
if let body = dictionary {
data = try? JSONSerialization.data(withJSONObject: body, options: .prettyPrinted)
}
let response = StubbedResponse(data: data, statusCode: statusCode)
var stubbedReponses = responses[path] ?? [StubbedResponse]()
stubbedReponses.append(response)
responses[path] = stubbedReponses
}
/// Append a body with status code to a given path. When calling this method multiple times for the same path
/// these stubbed requests will be triggered sequentially.
///
/// - Parameters:
/// - path: The path of the request, should be prefixid with "/"
/// - statusCode: The stubbed status code
/// - body: The stubbed JSON body code
internal func append(path: String, statusCode: Int, data: Data?) {
let response = StubbedResponse(data: data, statusCode: statusCode)
var stubbedReponses = responses[path] ?? [StubbedResponse]()
stubbedReponses.append(response)
responses[path] = stubbedReponses
}
/// Removes the stub for the given path and returns it. When there are multiple responses for the path we will
/// remove and return the first response.
///
/// - Parameters:
/// - path: The path of the request, should be prefixid with "/"
/// - Returns: The stubbed reponse if found
internal func removeStub(for path: String?) -> StubbedResponse? {
var pathNoSlash = path
pathNoSlash?.removeFirst()
guard let path = pathNoSlash else { return nil }
if self.responses[path]?.count ?? 0 > 0 {
return self.responses[path]?.removeFirst()
} else {
self.responses.removeValue(forKey: path)
return nil
}
}
/// Remove all stubbed responses.
private func removeAllStubs() {
responses.removeAll()
}
// MARK: - Fetching
/// Fetch stubbed reponses linked to a given path.
///
/// - Parameters:
/// - path: The path of the request, should be prefixid with "/"
/// - Returns: The stubbed reponses if found
internal subscript(path: String?) -> [StubbedResponse]? {
guard let path = path else { return nil }
return responses.fetch(for: path)
}
}
private extension Dictionary where Key == String {
func fetch(for path: String?) -> Value? {
guard
let path = path,
let key = keys.filter({ path.hasSuffix($0) }).first else {
return nil
}
return self[key]
}
}
| mit | 36cb5b61e8717afa88303cc20c418aa9 | 32.046875 | 115 | 0.622695 | 4.684385 | false | false | false | false |
JohnCoates/Aerial | Aerial/Source/Models/Cache/Cache.swift | 1 | 32068 | //
// Cache.swift
// Aerial
//
// Created by Guillaume Louel on 06/06/2020.
// Copyright © 2020 Guillaume Louel. All rights reserved.
//
import Cocoa
import CoreWLAN
import AVKit
/**
Aerial's new Cache management
Everything Cache related is moved here.
- Note: Where is our cache ?
Starting with 2.0, Aerial is putting its files in two locations :
- `~/Library/Application Support/Aerial/` : Contains manifests files and strings bundles for each source, in their own directory
- `~/Library/Application Support/Aerial/Cache/` : Contains (only) the cached videos
Users of version 1.x.x will automatically see their video files migrated to the correct location.
In Catalina, those paths live inside a user's container :
`~/Library/Containers/com.apple.ScreenSaver.Engine.legacyScreenSaver/Data/Library/Application Support/`
- Attention: Shared by multiple users writable locations are no longer possible, because sandboxing is awesome !
*/
// swiftlint:disable:next type_body_length
struct Cache {
/**
Returns the SSID of the Wi-Fi network the user is currently connected to.
- Note: Returns an empty string if not connected to Wi-Fi
*/
static var ssid: String {
return CWWiFiClient.shared().interface(withName: nil)?.ssid() ?? ""
}
static var processedSupportPath = ""
/**
Returns Aerial's Application Support path.
+ On macOS 10.14 and earlier : `~/Library/Application Support/Aerial/`
+ Starting with 10.15 : `~/Library/Containers/com.apple.ScreenSaver.Engine.legacyScreenSaver/Data/Library/Application Support/Aerial/`
- Note: Returns `/` on failure.
In some rare instances those system folders may not exist in the container, in this case Aerial can't work.
*/
static var supportPath: String {
// Dont' redo the thing all the time
if processedSupportPath != "" {
return processedSupportPath
}
var appPath = ""
if PrefsCache.overrideCache {
debugLog("Cache Override")
if !Aerial.helper.underCompanion, #available(macOS 12, *) {
if let bookmarkData = PrefsCache.supportBookmarkData {
do {
var isStale = false
let bookmarkUrl = try URL(resolvingBookmarkData: bookmarkData, options: .withSecurityScope, relativeTo: nil, bookmarkDataIsStale: &isStale)
//debugLog("Bookmark is stale : \(isStale)")
appPath = bookmarkUrl.path
do {
let url = try NSURL.init(resolvingBookmarkData: bookmarkData, options: .withoutUI, relativeTo: nil, bookmarkDataIsStale: nil)
url.startAccessingSecurityScopedResource()
} catch let error as NSError {
errorLog("Bookmark Access Failed: \(error.description)")
}
} catch let error {
errorLog("Can't process bookmark \(error)")
}
} else {
errorLog("Can't find supportBookmarkData on macOS 12")
}
} else {
if let customPath = PrefsCache.supportPath {
debugLog("Trying \(customPath)")
if FileManager.default.fileExists(atPath: customPath) {
appPath = customPath
} else {
errorLog("Could not find your custom Caches path, reverting to default settings")
}
} else {
errorLog("Empty path, reverting to default settings")
}
}
}
// This is the normal(ish) path
if appPath == "" {
// This is the normal path via screensaver
if !Aerial.helper.underCompanion {
// Grab an array of Application Support paths
let appSupportPaths = NSSearchPathForDirectoriesInDomains(
.applicationSupportDirectory,
.userDomainMask,
true)
if appSupportPaths.isEmpty {
errorLog("FATAL : app support does not exist!")
return "/"
}
appPath = appSupportPaths[0]
} else {
// If we are underCompanion, we need to add the container on 10.15+
// Grab an array of Application Support paths
if #available(OSX 10.15, *) {
let libPaths = NSSearchPathForDirectoriesInDomains(
.libraryDirectory,
.userDomainMask,
true)
appPath = libPaths.first! + "/Containers/com.apple.ScreenSaver.Engine.legacyScreenSaver/Data/Library/Application Support/"
} else {
let appSupportPaths = NSSearchPathForDirectoriesInDomains(
.applicationSupportDirectory,
.userDomainMask,
true)
if appSupportPaths.isEmpty {
errorLog("FATAL : app support does not exist!")
return "/"
}
appPath = appSupportPaths[0]
}
}
}
let appSupportDirectory = appPath as NSString
if aerialFolderExists(at: appSupportDirectory) {
processedSupportPath = appSupportDirectory.appendingPathComponent("Aerial")
return processedSupportPath
} else {
debugLog("Creating app support directory...")
let asPath = appSupportDirectory.appendingPathComponent("Aerial")
let fileManager = FileManager.default
do {
try fileManager.createDirectory(atPath: asPath,
withIntermediateDirectories: true, attributes: nil)
processedSupportPath = asPath
return asPath
} catch let error {
errorLog("FATAL : Couldn't create app support directory in User directory: \(error)")
return "/"
}
}
}
/**
Returns Aerial's Caches path.
+ On macOS 10.14 and earlier : `~/Library/Application Support/Aerial/Cache/`
+ Starting with 10.15 : `~/Library/Containers/com.apple.ScreenSaver.Engine.legacyScreenSaver/Data/Library/Application Support/Aerial/Cache/`
- Note: Returns `/` on failure.
In some rare instances those system folders may not exist in the container, in this case Aerial can't work.
Also note that the shared `Caches` folder, `/Library/Caches/Aerial/`, is no longer user writable in Catalina and will be ignored.
*/
static var path: String = {
var path = ""
/*if PrefsCache.overrideCache {
if #available(macOS 12, *) {
if let bookmarkData = PrefsCache.cacheBookmarkData {
do {
var isStale = false
let bookmarkUrl = try URL(resolvingBookmarkData: bookmarkData, options: .withSecurityScope, relativeTo: nil, bookmarkDataIsStale: &isStale)
debugLog("Bookmark is stale : \(isStale)")
debugLog("\(bookmarkUrl)")
path = bookmarkUrl.path
debugLog("\(path)")
} catch {
errorLog("Can't process bookmark")
}
} else {
errorLog("Can't find cacheBookmarkData on macOS 12")
}
} else {
if let customPath = Preferences.sharedInstance.customCacheDirectory {
debugLog("Trying \(customPath)")
if FileManager.default.fileExists(atPath: customPath) {
path = customPath
} else {
errorLog("Could not find your custom Caches path, reverting to default settings")
}
} else {
errorLog("Empty path, reverting to default settings")
}
}
if path == "" {
PrefsCache.overrideCache = false
path = Cache.supportPath.appending("/Cache")
}
} else {*/
path = Cache.supportPath.appending("/Cache")
// }
if FileManager.default.fileExists(atPath: path as String) {
return path
} else {
do {
try FileManager.default.createDirectory(atPath: path,
withIntermediateDirectories: true, attributes: nil)
return path
} catch let error {
errorLog("FATAL : Couldn't create Cache directory in Aerial's AppSupport directory: \(error)")
return "/"
}
}
}()
static var pathUrl: URL = {
if #available(macOS 12, *) {
if PrefsCache.overrideCache {
if let bookmarkData = PrefsCache.cacheBookmarkData {
do {
var isStale = false
let bookmarkUrl = try URL(resolvingBookmarkData: bookmarkData, options: .withSecurityScope, relativeTo: nil, bookmarkDataIsStale: &isStale)
debugLog("Bookmark is stale : \(isStale)")
debugLog("\(bookmarkUrl)")
return bookmarkUrl
} catch {
errorLog("Can't process bookmark")
}
}
}
}
return URL(fileURLWithPath: path)
}()
/**
Returns Aerial's thumbnail cache path, creating it if needed.
+ On macOS 10.14 and earlier : `~/Library/Application Support/Aerial/Thumbnails/`
+ Starting with 10.15 : `~/Library/Containers/com.apple.ScreenSaver.Engine.legacyScreenSaver/Data/Library/Application Support/Aerial/Thumbnails/`
- Note: Returns `/` on failure.
*/
static var thumbnailsPath: String = {
let path = Cache.supportPath.appending("/Thumbnails")
if FileManager.default.fileExists(atPath: path as String) {
return path
} else {
do {
try FileManager.default.createDirectory(atPath: path,
withIntermediateDirectories: true, attributes: nil)
return path
} catch let error {
errorLog("FATAL : Couldn't create Thumbnails directory in Aerial's AppSupport directory: \(error)")
return "/"
}
}
}()
/**
Returns Aerial's former cache path, if it exists.
+ On macOS 10.14 and earlier : `~/Library/Caches/Aerial/`
+ Starting with 10.15 : `~/Library/Containers/com.apple.ScreenSaver.Engine.legacyScreenSaver/Data/Library/Caches/Aerial/`
- Note: Returns `nil` on failure.
*/
static private var formerCachePath: String? = {
// Grab an array of Cache paths
let cacheSupportPaths = NSSearchPathForDirectoriesInDomains(
.cachesDirectory,
.userDomainMask,
true)
if cacheSupportPaths.isEmpty {
errorLog("Couldn't find Caches paths!")
return nil
}
let cacheSupportDirectory = cacheSupportPaths[0] as NSString
if aerialFolderExists(at: cacheSupportDirectory) {
return cacheSupportDirectory.appendingPathComponent("Aerial")
} else {
do {
debugLog("trying to create \(cacheSupportDirectory.appendingPathComponent("Aerial"))")
try FileManager.default.createDirectory(atPath: cacheSupportDirectory.appendingPathComponent("Aerial"),
withIntermediateDirectories: true, attributes: nil)
return path
} catch {
errorLog("Could not create Aerial's Caches path")
}
return nil
}
}()
// MARK: - Migration from Aerial 1.x.x to 2.x.x
/**
Migrate files from previous versions of Aerial to the 2.x.x structure.
- Moves the video files from Application Support to the `Application Support/Aerial/Cache` sub directory.
- Moves the video files from Caches to the `Application Support/Aerial/Cache` sub directory
*/
static func migrate() {
if !PrefsCache.overrideCache {
migrateAppSupport()
migrateOldCache()
}
}
/**
Migrate video that may be at the root of /Application Support/Aerial/
*/
static private func migrateAppSupport() {
let supportURL = URL(fileURLWithPath: supportPath as String)
do {
let directoryContent = try FileManager.default.contentsOfDirectory(at: supportURL, includingPropertiesForKeys: nil)
let videoURLs = directoryContent.filter { $0.pathExtension == "mov" }
if !videoURLs.isEmpty {
debugLog("Starting migration of your video files from Application Support to the /Cache subfolder")
for videoURL in videoURLs {
debugLog("moving \(videoURL.lastPathComponent)")
let newURL = URL(fileURLWithPath: path.appending("/\(videoURL.lastPathComponent)"))
try FileManager.default.moveItem(at: videoURL, to: newURL)
}
debugLog("Migration done.")
}
} catch {
errorLog("Error during migration, please report")
errorLog(error.localizedDescription)
}
}
/**
Migrate video that may be at the root of a user's /Caches/Aerial/
*/
static private func migrateOldCache() {
if let formerCachePath = formerCachePath {
do {
let formerCacheURL = URL(fileURLWithPath: formerCachePath as String)
let directoryContent = try FileManager.default.contentsOfDirectory(at: formerCacheURL, includingPropertiesForKeys: nil)
let videoURLs = directoryContent.filter { $0.pathExtension == "mov" }
if !videoURLs.isEmpty {
debugLog("Starting migration of your video files from Caches to the /Cache subfolder of Application Support")
for videoURL in videoURLs {
debugLog("moving \(videoURL.lastPathComponent)")
let newURL = URL(fileURLWithPath: path.appending("/\(videoURL.lastPathComponent)"))
try FileManager.default.moveItem(at: videoURL, to: newURL)
}
debugLog("Migration done.")
}
} catch {
errorLog("Error during migration, please report")
errorLog(error.localizedDescription)
}
}
}
// Remove files in bad format or outdated
static func removeCruft() {
// TODO: kind of a temporary safety
if VideoList.instance.videos.count > 90 {
// First let's look at the cache
// let pathURL = URL(fileURLWithPath: path)
do {
pathUrl.startAccessingSecurityScopedResource()
let directoryContent = try FileManager.default.contentsOfDirectory(at: pathUrl, includingPropertiesForKeys: nil)
debugLog("count : \(directoryContent.count)")
let videoURLs = directoryContent.filter { $0.pathExtension == "mov" }
for video in videoURLs {
let filename = video.lastPathComponent
debugLog("\(filename)")
var found = false
// swiftlint:disable for_where
for candidate in VideoList.instance.videos {
if candidate.url.lastPathComponent == filename {
found = true
}
}
if !found {
debugLog("This file is not in the correct format or outdated, removing : \(video)")
try? FileManager.default.removeItem(at: video)
}
}
pathUrl.stopAccessingSecurityScopedResource()
} catch {
errorLog("Error during removing of videos in wrong format, please report")
errorLog(error.localizedDescription)
}
// Also remove uncached cruft
removeUncachedCruft()
}
}
static func removeUncachedCruft() {
for source in SourceList.foundSources where !source.isCachable && source.type != .local {
debugLog("Checking cruft in \(source.name)")
let pathURL = URL(fileURLWithPath: supportPath.appending("/" + source.name))
let unprocessed = source.getUnprocessedVideos()
debugLog(pathURL.absoluteString)
do {
let directoryContent = try FileManager.default.contentsOfDirectory(at: pathURL, includingPropertiesForKeys: nil)
let videoURLs = directoryContent.filter { $0.pathExtension == "mov" }
for video in videoURLs {
let filename = video.lastPathComponent
var found = false
// swiftlint:disable for_where
for candidate in unprocessed {
if candidate.url.lastPathComponent == filename {
found = true
}
}
if !found {
debugLog("This file is not in the correct format or outdated, removing : \(video)")
try? FileManager.default.removeItem(at: video)
}
}
} catch {
errorLog("Error during removal of videos in wrong format, please report")
errorLog(error.localizedDescription)
}
}
}
/// This clears the whole cache. User beware !
static func clearCache() {
let pathURL = URL(fileURLWithPath: path)
do {
let directoryContent = try FileManager.default.contentsOfDirectory(at: pathURL, includingPropertiesForKeys: nil)
let videoURLs = directoryContent.filter { $0.pathExtension == "mov" }
for video in videoURLs {
try? FileManager.default.removeItem(at: video)
}
} catch {
errorLog("Error during removal of videos in wrong format, please report")
errorLog(error.localizedDescription)
}
}
static func clearNonCacheableSources() {
// Then we need to look at individual online sources
// let onlineVideos = VideoList.instance.videos.filter({ !$0.source.isCachable })
for source in SourceList.foundSources.filter({!$0.isCachable}) {
let pathSource = URL(fileURLWithPath: supportPath).appendingPathComponent(source.name)
if FileManager.default.fileExists(atPath: pathSource.path) {
do {
let directoryContent = try FileManager.default.contentsOfDirectory(at: pathSource, includingPropertiesForKeys: nil)
let videoURLs = directoryContent.filter { $0.pathExtension == "mov" }
for video in videoURLs {
debugLog("Removing file : \(video)")
try? FileManager.default.removeItem(at: video)
}
} catch {
errorLog("Error during removing of videos in wrong format, please report")
errorLog(error.localizedDescription)
}
}
}
}
// MARK: - About the cache
/**
Is our cache full ?
*/
static func isFull() -> Bool {
return size() > PrefsCache.cacheLimit
}
/**
Do we still have a bit of free space (0.5 GB)
*/
static func hasSomeFreeSpace() -> Bool {
return size() < PrefsCache.cacheLimit - 0.5
}
/**
Returns the cache size in GB as a string (eg. 5.1 GB)
*/
static func sizeString() -> String {
let pathURL = Foundation.URL(fileURLWithPath: path)
// check if the url is a directory
if (try? pathURL.resourceValues(forKeys: [.isDirectoryKey]))?.isDirectory == true {
var folderSize = 0
(FileManager.default.enumerator(at: pathURL, includingPropertiesForKeys: nil)?.allObjects as? [URL])?.lazy.forEach {
folderSize += (try? $0.resourceValues(forKeys: [.totalFileAllocatedSizeKey]))?.totalFileAllocatedSize ?? 0
}
let byteCountFormatter = ByteCountFormatter()
byteCountFormatter.allowedUnits = .useGB
byteCountFormatter.countStyle = .file
let sizeToDisplay = byteCountFormatter.string(for: folderSize) ?? ""
return sizeToDisplay
}
// In case it fails somehow
return "No cache found"
}
// MARK: - Helpers
/**
Does an `/Aerial/` subfolder exist inside the given path
- parameter at: Source path
- returns: Path existance as a Bool.
*/
private static func aerialFolderExists(at: NSString) -> Bool {
let aerialFolder = at.appendingPathComponent("Aerial")
return FileManager.default.fileExists(atPath: aerialFolder as String)
}
/**
Returns cache size in GB
*/
static func size() -> Double {
let pathURL = URL(fileURLWithPath: path)
// check if the url is a directory
if (try? pathURL.resourceValues(forKeys: [.isDirectoryKey]))?.isDirectory == true {
var folderSize = 0
(FileManager.default.enumerator(at: pathURL, includingPropertiesForKeys: nil)?.allObjects as? [URL])?.lazy.forEach {
folderSize += (try? $0.resourceValues(forKeys: [.totalFileAllocatedSizeKey]))?.totalFileAllocatedSize ?? 0
}
return Double(folderSize) / 1000000000
}
return 0
}
static func getDirectorySize(directory: String) -> Double {
if FileManager.default.fileExists(atPath: directory) {
let pathURL = URL(fileURLWithPath: directory)
// check if the url is a directory
if (try? pathURL.resourceValues(forKeys: [.isDirectoryKey]))?.isDirectory == true {
var folderSize = 0
(FileManager.default.enumerator(at: pathURL, includingPropertiesForKeys: nil)?.allObjects as? [URL])?.lazy.forEach {
folderSize += (try? $0.resourceValues(forKeys: [.totalFileAllocatedSizeKey]))?.totalFileAllocatedSize ?? 0
}
return Double(folderSize) / 1000000000
}
return 0
} else {
return 0
}
}
static func packsSize() -> Double {
var totalSize: Double = 0
for source in SourceList.foundSources where !source.isCachable {
let sourcePath = supportPath.appending("/" + source.name)
totalSize += getDirectorySize(directory: sourcePath)
}
return totalSize
}
// swiftlint:disable line_length
// MARK: Networking restrictions for cache
/**
Can we download a file ?
Depending on user's settings, the cache may be full or the user may not be on a trusted network.
- Note: If a user disabled cache management (full manual mode), this will always be true.
- parameter action: A closure with the action to be accomplished should the conditions be met.
*/
static func ensureDownload(action: @escaping () -> Void) {
// Do we manage the cache or not ?
if PrefsCache.enableManagement {
// Check network first
if !canNetwork() {
if !Aerial.helper.showAlert(question: "You are on a restricted WiFi network",
text: "Your current settings restrict downloads when not connected to a trusted network. Do you wish to proceed?\n\nReminder: You can change this setting in the Cache tab.",
button1: "Download Anyway",
button2: "Cancel") {
return
}
}
// Then cache status
if isFull() {
let formatter = NumberFormatter()
formatter.locale = Locale.current // USA: Locale(identifier: "en_US")
formatter.numberStyle = .decimal
let result = formatter.string(from: NSNumber(value: PrefsCache.cacheLimit.rounded(toPlaces: 1)))!
print(result) // -> US$9,999.99
if !Aerial.helper.showAlert(question: "Your cache is full",
text: "Your cache limit is currently set to \(result) GB, and currently contains \(Cache.sizeString()) of files.\n\n Do you want to proceed with the download anyway?\n\nYou can manually increase or decrease your cache size in Settings > Cache.",
button1: "Download Anyway",
button2: "Cancel") {
return
}
}
}
// If all is fine then proceed
action()
}
/**
Can we safely use network ?
Depending on user's settings, they may not be on a trusted network.
- Note: If a user disabled cache management (full manual mode), this will always be true.
*/
static func canNetwork() -> Bool {
if !PrefsCache.enableManagement {
return true
}
if PrefsCache.restrictOnWiFi {
// If we are not connected to WiFi we allow
if Cache.ssid == "" || PrefsCache.allowedNetworks.contains(ssid) {
return true
} else {
return false
}
} else {
return true
}
}
static func outdatedVideos() -> [AerialVideo] {
guard PrefsCache.enableManagement else {
return []
}
var cutoffDate = Date()
switch PrefsCache.cachePeriodicity {
case .daily:
cutoffDate = Calendar.current.date(byAdding: .day, value: -1, to: cutoffDate)!
case .weekly:
cutoffDate = Calendar.current.date(byAdding: .day, value: -7, to: cutoffDate)!
case .monthly:
cutoffDate = Calendar.current.date(byAdding: .month, value: -1, to: cutoffDate)!
case .never:
return []
}
// Get a list of cached videos that are not favorites, and are from a cacheable source (not a pack)
// Yes this is getting a bit complicated
var evictable: [Date: AerialVideo] = [:]
let currentlyCached = VideoList.instance.videos.filter({ $0.isAvailableOffline && $0.source.isCachable && !PrefsVideos.favorites.contains($0.id)})
for video in currentlyCached {
let path = VideoCache.cachePath(forVideo: video)!
// swiftlint:disable:next force_try
let attributes = try! FileManager.default.attributesOfItem(atPath: path)
let creationDate = attributes[.creationDate] as! Date
if creationDate < cutoffDate {
evictable[creationDate] = video
}
}
return evictable.sorted { $0.key < $1.key }.map({ $0.value })
}
// swiftlint:disable:next cyclomatic_complexity
static func freeCache() {
guard PrefsCache.enableManagement else {
return
}
// Step 1 : Delete hidden videos
debugLog("Looking for hidden videos to delete...")
for video in VideoList.instance.videos.filter({ PrefsVideos.hidden.contains($0.id) && $0.isAvailableOffline }) {
debugLog("Deleting hidden video \(video.secondaryName)")
do {
let path = VideoList.instance.localPathFor(video: video)
try FileManager.default.removeItem(atPath: path)
} catch {
errorLog("Could not delete video : \(video.secondaryName)")
}
}
// We may be good ?
if hasSomeFreeSpace() {
return
}
// Step 2 : Delete videos that are out of rotation
let evictables = outdatedVideos()
if evictables.isEmpty {
debugLog("No outdated videos, we won't delete anything")
return
}
debugLog("Looking for outdated videos that aren't in rotation (candidates : \(evictables.count)")
outerLoop: for video in evictables {
if VideoList.instance.currentRotation().contains(video) {
// Outdated but in rotation, so keep it !
// debugLog("outdated but in rotation \(video.secondaryName)")
} else {
debugLog("Removing outdated video not in rotation \(video.secondaryName)")
do {
try FileManager.default.removeItem(atPath: VideoCache.cachePath(forVideo: video)!)
} catch {
errorLog("Could not delete video : \(video.secondaryName)")
}
if hasSomeFreeSpace() {
// Removed enough
break outerLoop
}
}
}
// Are we there yeeeet ?
if hasSomeFreeSpace() {
return
}
debugLog("Looking for outdated videos that may still be in rotation (candidates : \(evictables.count)")
var currentVideos = [AerialVideo]()
for view in AerialView.instanciatedViews {
if let video = view.currentVideo {
currentVideos.append(video)
}
}
outerLoop2: for video in evictables {
if currentVideos.contains(video) {
debugLog("\(video.secondaryName) is currently playing, trying another")
} else {
debugLog("Removing outdated video that was in rotation \(video.secondaryName)")
do {
try FileManager.default.removeItem(atPath: VideoCache.cachePath(forVideo: video)!)
} catch {
errorLog("Could not delete video : \(video.secondaryName)")
}
if hasSomeFreeSpace() {
// Removed enough
break outerLoop2
}
}
}
// At this point we can't do more
}
static func fillOrRollCache() {
guard PrefsCache.enableManagement && canNetwork() else {
return
}
// Grab a *shuffled* list of uncached in rotation videos
let rotation = VideoList.instance.currentRotation().filter { !$0.isAvailableOffline }.shuffled()
if rotation.isEmpty {
debugLog("> Current playlist is already fully cached, no download/rotation needed")
return
}
debugLog("> Fill or roll cache")
// Do we have some space to download at least a video (by default .5 GB) ?
if !hasSomeFreeSpace() {
freeCache()
if !hasSomeFreeSpace() {
debugLog("No free space to reclaim currently.")
return
}
}
debugLog("Uncached videos in rotation : \(rotation.count)")
// We may be satisfied already
if rotation.isEmpty {
return
}
// Queue the first video on the list
debugLog("Queuing video : \(rotation.first!.secondaryName)")
VideoManager.sharedInstance.queueDownload(rotation.first!)
}
}
| mit | ede2c70c75b20ae2f21921f1a146ea66 | 37.916262 | 289 | 0.560015 | 5.632707 | false | false | false | false |
YoungGary/30daysSwiftDemo | day21/day21/ViewController.swift | 1 | 3157 | //
// ViewController.swift
// day21
//
// Created by YOUNG on 2016/10/15.
// Copyright © 2016年 Young. All rights reserved.
//
import UIKit
class ViewController: UIViewController,UITableViewDataSource,UITableViewDelegate{
@IBOutlet weak var tableView: UITableView!
let datas : [Model] = [Model(image : "1",title:"1"),Model(image : "2",title:"2"),Model(image : "3",title:"3"),Model(image : "4",title:"4"),Model(image : "5",title:"5"),Model(image : "6",title:"6"),Model(image : "7",title:"7"),Model(image : "8",title:"8"),Model(image : "1",title:"1"),Model(image : "2",title:"2"),Model(image : "3",title:"3"),Model(image : "4",title:"4"),Model(image : "5",title:"5"),Model(image : "6",title:"6"),Model(image : "7",title:"7"),Model(image : "8",title:"8")]
override func viewDidLoad() {
super.viewDidLoad()
tableView.dataSource = self
tableView.delegate = self
tableView.tableFooterView = UIView()
tableView.contentInset = UIEdgeInsetsMake(-64, 0, 0, 0);
//nib
let nib = UINib(nibName: "TableViewCell", bundle: nil)
tableView.registerNib(nib, forCellReuseIdentifier: "cell")
}
func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return datas.count
}
func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCellWithIdentifier("cell", forIndexPath: indexPath) as! TableViewCell
cell.model = datas[indexPath.row]
return cell
}
func tableView(tableView: UITableView, heightForRowAtIndexPath indexPath: NSIndexPath) -> CGFloat {
return 80
}
//delegate --- editing
func tableView(tableView: UITableView, canEditRowAtIndexPath indexPath: NSIndexPath) -> Bool {
return true
}
func tableView(tableView: UITableView, editActionsForRowAtIndexPath indexPath: NSIndexPath) -> [UITableViewRowAction]?{
let delete = UITableViewRowAction(style: .Normal, title: "🗑\nDelete") { action, index in
print("Delete button tapped")
}
delete.backgroundColor = UIColor.grayColor()
let share = UITableViewRowAction(style: .Normal, title: "🤗\nShare") { (action: UITableViewRowAction!, indexPath: NSIndexPath) -> Void in
let firstActivityItem = self.datas[indexPath.row]
let activityViewController = UIActivityViewController(activityItems: [firstActivityItem.image as NSString], applicationActivities: nil)
self.presentViewController(activityViewController, animated: true, completion: nil)
}
share.backgroundColor = UIColor.redColor()
let download = UITableViewRowAction(style: .Normal, title: "⬇️\nDownload") { action, index in
print("Download button tapped")
}
download.backgroundColor = UIColor.blueColor()
return [download, share, delete]
}
}
| mit | 2052c8c2fb11804c91d545cd14fba396 | 33.549451 | 491 | 0.629771 | 4.792683 | false | false | false | false |
yunzixun/V2ex-Swift | Controller/MoreViewController.swift | 1 | 3462 | //
// MoreViewController.swift
// V2ex-Swift
//
// Created by huangfeng on 1/30/16.
// Copyright © 2016 Fin. All rights reserved.
//
import UIKit
class MoreViewController: UITableViewController {
override func viewDidLoad() {
super.viewDidLoad()
self.title = NSLocalizedString("more")
self.tableView.separatorStyle = UITableViewCellSeparatorStyle.none;
regClass(self.tableView, cell: BaseDetailTableViewCell.self)
self.themeChangedHandler = {[weak self] (style) -> Void in
self?.view.backgroundColor = V2EXColor.colors.v2_backgroundColor
self?.tableView.reloadData()
}
}
override func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int{
return 9
}
override func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
return [30,50,12,50,50,12,50,50,50][indexPath.row]
}
override func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = getCell(tableView, cell: BaseDetailTableViewCell.self, indexPath: indexPath)
cell.selectionStyle = .none
//设置标题
cell.titleLabel.text = [
"",
NSLocalizedString("viewOptions"),
"",
NSLocalizedString("rateV2ex"),
NSLocalizedString("reportAProblem"),
"",
NSLocalizedString("followThisProjectSourceCode"),
NSLocalizedString("open-SourceLibraries"),
NSLocalizedString("version")][indexPath.row]
//设置颜色
if [0,2,5].contains(indexPath.row) {
cell.backgroundColor = self.tableView.backgroundColor
}
else{
cell.backgroundColor = V2EXColor.colors.v2_CellWhiteBackgroundColor
}
//设置右侧箭头
if [0,2,5,8].contains(indexPath.row) {
cell.detailMarkHidden = true
}
else {
cell.detailMarkHidden = false
}
//设置右侧文本
if indexPath.row == 8 {
cell.detailLabel.text = "Version " + (Bundle.main.infoDictionary!["CFBundleShortVersionString"] as! String)
+ " (Build " + (Bundle.main.infoDictionary!["CFBundleVersion"] as! String ) + ")"
}
else {
cell.detailLabel.text = ""
}
return cell
}
override func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
if indexPath.row == 1 {
V2Client.sharedInstance.centerNavigation?.pushViewController(SettingsTableViewController(), animated: true)
}
else if indexPath.row == 3 {
let str = "itms-apps://itunes.apple.com/WebObjects/MZStore.woa/wa/viewSoftware?id=1078157349"
UIApplication.shared.openURL(URL(string: str)!)
}
else if indexPath.row == 4 {
UIApplication.shared.openURL(URL(string: "https://day.app/2016/02/v2ex-ioske-hu-duan-bug-and-jian-yi/")!)
}
else if indexPath.row == 6 {
UIApplication.shared.openURL(URL(string: "https://github.com/Finb/V2ex-Swift")!)
}
else if indexPath.row == 7 {
V2Client.sharedInstance.centerNavigation?.pushViewController(PodsTableViewController(), animated: true)
}
}
}
| mit | 4463dbf83e0db3324b4c216a6d20dcca | 35.010526 | 119 | 0.603332 | 4.764624 | false | false | false | false |
Sadmansamee/quran-ios | Quran/DefaultQuranImageService.swift | 1 | 3342 | //
// DefaultQuranImageService.swift
// Quran
//
// Created by Mohamed Afifi on 5/2/16.
// Copyright © 2016 Quran.com. All rights reserved.
//
import UIKit
class DefaultQuranImageService: QuranImageService {
let imagesCache: Cache<Int, UIImage>
fileprivate let preloadPreviousImagesCount = 1
fileprivate let preloadNextImagesCount = 2
fileprivate let queue = OperationQueue()
init(imagesCache: Cache<Int, UIImage>) {
self.imagesCache = imagesCache
}
fileprivate var inProgressOperations: [Int: ImagePreloadingOperation] = [:]
fileprivate let lock = NSLock()
internal func getImageOfPage(_ page: Int, forSize size: CGSize, onCompletion: @escaping (UIImage) -> Void) {
let image = lock.execute { imagesCache.object(forKey: page) }
if let image = image as UIImage! {
onCompletion(image)
// schedule for close images
cachePagesCloserToPage(page)
return
}
// preload requested page with very high priority and QoS
preload(page, priority: .veryHigh, qualityOfService: .userInitiated) { (page, image) in
Queue.main.async {
onCompletion(image)
}
}
// schedule for close images
cachePagesCloserToPage(page)
}
fileprivate func cachePagesCloserToPage(_ page: Int) {
// load next pages
for index in 0..<preloadNextImagesCount {
let targetPage = page + 1 + index
if !((imagesCache.object(forKey: targetPage) != nil)) {
preload(targetPage, onCompletion: { _ in })
}
}
// load previous pages
for index in 0..<preloadPreviousImagesCount {
let targetPage = page - 1 - index
if !((imagesCache.object(forKey: targetPage) != nil)) {
preload(targetPage, onCompletion: { _ in })
}
}
}
func preload(_ page: Int,
priority: Operation.QueuePriority = .normal,
qualityOfService: QualityOfService = .background,
onCompletion: @escaping (Int, UIImage) -> Void) {
guard Quran.QuranPagesRange.contains(page) else {
return // does nothing
}
lock.execute {
if let operation = inProgressOperations[page] {
operation.addCompletionBlock(onCompletion)
} else {
// create the operation
let operation = ImagePreloadingOperation(page: page)
// cache the result
operation.addCompletionBlock { [weak self] page, image in
Queue.main.async {
self?.lock.execute {
// cache the image
self?.imagesCache.setObject(image, forKey: page)
// remove from in progress
_ = self?.inProgressOperations.removeValue(forKey: page)
}
}
}
// on complete
operation.addCompletionBlock(onCompletion)
// add it to the in progress
inProgressOperations[page] = operation
queue.addOperation(operation)
}
}
}
}
| mit | 2c9811ea236f09a73721f45925e84cb8 | 30.819048 | 112 | 0.555223 | 5.354167 | false | false | false | false |
RedMadRobot/input-mask-ios | Source/InputMask/InputMaskTests/Classes/Mask/WildcardCase.swift | 1 | 8880 | //
// Project «InputMask»
// Created by Jeorge Taflanidi
//
import XCTest
@testable import InputMask
class WildcardCase: MaskTestCase {
override func format() -> String {
return "[…]"
}
func testInit_correctFormat_maskInitialized() {
XCTAssertNotNil(try self.mask())
}
func testInit_correctFormat_measureTime() {
self.measure {
var masks: [Mask] = []
for _ in 1...1000 {
masks.append(
try! self.mask()
)
}
}
}
func testGetOrCreate_correctFormat_measureTime() {
self.measure {
var masks: [Mask] = []
for _ in 1...1000 {
masks.append(
try! Mask.getOrCreate(withFormat: self.format())
)
}
}
}
func testGetPlaceholder_allSet_returnsCorrectPlaceholder() {
let placeholder: String = try! self.mask().placeholder
XCTAssertEqual(placeholder, "")
}
func testAcceptableTextLength_allSet_returnsCorrectCount() {
let acceptableTextLength: Int = try! self.mask().acceptableTextLength
XCTAssertEqual(acceptableTextLength, 1)
}
func testTotalTextLength_allSet_returnsCorrectCount() {
let totalTextLength: Int = try! self.mask().totalTextLength
XCTAssertEqual(totalTextLength, 1)
}
func testAcceptableValueLength_allSet_returnsCorrectCount() {
let acceptableValueLength: Int = try! self.mask().acceptableValueLength
XCTAssertEqual(acceptableValueLength, 1)
}
func testTotalValueLength_allSet_returnsCorrectCount() {
let totalValueLength: Int = try! self.mask().totalValueLength
XCTAssertEqual(totalValueLength, 1)
}
func testApply_emptyString_returns_Complete() {
let inputString: String = ""
let inputCaret: String.Index = inputString.endIndex
let expectedString: String = ""
let expectedCaret: String.Index = expectedString.endIndex
let expectedValue: String = expectedString
let result: Mask.Result = try! self.mask().apply(
toText: CaretString(
string: inputString,
caretPosition: inputCaret,
caretGravity: .forward(autocomplete: false)
)
)
XCTAssertEqual(expectedString, result.formattedText.string)
XCTAssertEqual(expectedCaret, result.formattedText.caretPosition)
XCTAssertEqual(expectedValue, result.extractedValue)
XCTAssertEqual(true, result.complete)
}
func testApply_J_returns_J() {
let inputString: String = "J"
let inputCaret: String.Index = inputString.endIndex
let expectedString: String = "J"
let expectedCaret: String.Index = expectedString.endIndex
let expectedValue: String = expectedString
let result: Mask.Result = try! self.mask().apply(
toText: CaretString(
string: inputString,
caretPosition: inputCaret,
caretGravity: .forward(autocomplete: false)
)
)
XCTAssertEqual(expectedString, result.formattedText.string)
XCTAssertEqual(expectedCaret, result.formattedText.caretPosition)
XCTAssertEqual(expectedValue, result.extractedValue)
XCTAssertEqual(true, result.complete)
}
func testApply_Je_returns_Je() {
let inputString: String = "Je"
let inputCaret: String.Index = inputString.endIndex
let expectedString: String = "Je"
let expectedCaret: String.Index = expectedString.endIndex
let expectedValue: String = expectedString
let result: Mask.Result = try! self.mask().apply(
toText: CaretString(
string: inputString,
caretPosition: inputCaret,
caretGravity: .forward(autocomplete: false)
)
)
XCTAssertEqual(expectedString, result.formattedText.string)
XCTAssertEqual(expectedCaret, result.formattedText.caretPosition)
XCTAssertEqual(expectedValue, result.extractedValue)
XCTAssertEqual(true, result.complete)
}
func testApply_Jeo_returns_Jeo() {
let inputString: String = "Jeo"
let inputCaret: String.Index = inputString.endIndex
let expectedString: String = "Jeo"
let expectedCaret: String.Index = expectedString.endIndex
let expectedValue: String = expectedString
let result: Mask.Result = try! self.mask().apply(
toText: CaretString(
string: inputString,
caretPosition: inputCaret,
caretGravity: .forward(autocomplete: false)
)
)
XCTAssertEqual(expectedString, result.formattedText.string)
XCTAssertEqual(expectedCaret, result.formattedText.caretPosition)
XCTAssertEqual(expectedValue, result.extractedValue)
XCTAssertEqual(true, result.complete)
}
func testApply_Jeor_returns_Jeor() {
let inputString: String = "Jeor"
let inputCaret: String.Index = inputString.endIndex
let expectedString: String = "Jeor"
let expectedCaret: String.Index = expectedString.endIndex
let expectedValue: String = expectedString
let result: Mask.Result = try! self.mask().apply(
toText: CaretString(
string: inputString,
caretPosition: inputCaret,
caretGravity: .forward(autocomplete: false)
)
)
XCTAssertEqual(expectedString, result.formattedText.string)
XCTAssertEqual(expectedCaret, result.formattedText.caretPosition)
XCTAssertEqual(expectedValue, result.extractedValue)
XCTAssertEqual(true, result.complete)
}
func testApply_Jeorge_returns_Jeorge() {
let inputString: String = "Jeorge"
let inputCaret: String.Index = inputString.endIndex
let expectedString: String = "Jeorge"
let expectedCaret: String.Index = expectedString.endIndex
let expectedValue: String = expectedString
let result: Mask.Result = try! self.mask().apply(
toText: CaretString(
string: inputString,
caretPosition: inputCaret,
caretGravity: .forward(autocomplete: false)
)
)
XCTAssertEqual(expectedString, result.formattedText.string)
XCTAssertEqual(expectedCaret, result.formattedText.caretPosition)
XCTAssertEqual(expectedValue, result.extractedValue)
XCTAssertEqual(true, result.complete)
}
func testApply_Jeorge1_returns_Jeorge() {
let inputString: String = "Jeorge1"
let inputCaret: String.Index = inputString.endIndex
let expectedString: String = "Jeorge1"
let expectedCaret: String.Index = expectedString.endIndex
let expectedValue: String = expectedString
let result: Mask.Result = try! self.mask().apply(
toText: CaretString(
string: inputString,
caretPosition: inputCaret,
caretGravity: .forward(autocomplete: false)
)
)
XCTAssertEqual(expectedString, result.formattedText.string)
XCTAssertEqual(expectedCaret, result.formattedText.caretPosition)
XCTAssertEqual(expectedValue, result.extractedValue)
XCTAssertEqual(true, result.complete)
}
func testApply_1Jeorge2_returns_Jeorge() {
let inputString: String = "1Jeorge2"
let inputCaret: String.Index = inputString.endIndex
let expectedString: String = "1Jeorge2"
let expectedCaret: String.Index = expectedString.endIndex
let expectedValue: String = expectedString
let result: Mask.Result = try! self.mask().apply(
toText: CaretString(
string: inputString,
caretPosition: inputCaret,
caretGravity: .forward(autocomplete: false)
)
)
XCTAssertEqual(expectedString, result.formattedText.string)
XCTAssertEqual(expectedCaret, result.formattedText.caretPosition)
XCTAssertEqual(expectedValue, result.extractedValue)
XCTAssertEqual(true, result.complete)
}
}
| mit | b5ababfa298fbfacaaadb7ccae103a87 | 34.222222 | 79 | 0.597566 | 5.585903 | false | true | false | false |
itanchao/TCRunloopView | Sauces/RunLoopSwiftView.swift | 1 | 12371 | //
// TCRunLoopView.swift
// TCRunLoopView
//
// Created by tanchao on 16/4/19.
// Copyright © 2016年 谈超. All rights reserved.
//
import UIKit
import Foundation
import SDWebImage
public protocol TCRunLoopViewDelegate: NSObjectProtocol, UIScrollViewDelegate {
func runLoopViewDidClick(_ loopView:TCRunLoopView, didSelectRowAtIndex index: NSInteger)
func runLoopViewDidScroll(_ loopView: TCRunLoopView, didScrollRowAtIndex index: NSInteger)
}
public struct LoopData {
public var imageUrl : String = ""
public var desc : String = ""
public init(image:String = "",des:String = ""){
imageUrl = image
desc = des
}
// 序列化
public func serialize() -> [String : Any] {
return ["imageUrl":imageUrl ,"desc":desc]
}
}
// MARK:变量与控件
public class TCRunLoopView: UIView {
/// 代理
public var delegate : TCRunLoopViewDelegate?
/// 轮播间隔
public var loopInterval : TimeInterval = 5{
didSet{
assert(loopInterval > 0, "请设置一个大于0的数 the value of loopInterVal must be greater than 0")
if timer != nil {
self.stop()
}
timer = self.getTime()
self.runloopViewFire()
}
}
/// 轮播数据
public var loopDataGroup : [LoopData] = []{
didSet{
setUpRunloopView()
pageControl.numberOfPages = loopDataGroup.count
currIndex = 0
}
}
// 私有变量
fileprivate var currIndex : Int = 0{
didSet{
pageControl.currentPage = currIndex
delegate?.runLoopViewDidScroll(self, didScrollRowAtIndex: currIndex)
// if (delegate != nil) && (delegate?.responds(to: #selector(delegate?.runLoopViewDidScroll(_:didScrollRowAtIndex:))))! {
// delegate?.runLoopViewDidScroll!(self, didScrollRowAtIndex: currIndex)
// }
}
}
fileprivate var timer : Timer?
// MARK:懒加载控件
lazy fileprivate var scrollView: UIScrollView = {
let view = UIScrollView()
view.isPagingEnabled = true
view.delegate = self
view.showsHorizontalScrollIndicator = false
self.addSubview(view)
return view
}()
lazy fileprivate var pageControl: UIPageControl = {
let view = UIPageControl()
view.hidesForSinglePage = true
view.currentPageIndicatorTintColor = UIColor.white
view.pageIndicatorTintColor = UIColor.black
view.addTarget(self, action: #selector(TCRunLoopView.pageAction), for: .touchUpInside)
self.insertSubview(view, aboveSubview: self.scrollView)
return view
}()
lazy fileprivate var leftImageView: RunloopCell = {
let iconView = RunloopCell()
self.scrollView.addSubview(iconView)
return iconView
}()
lazy fileprivate var centerImageView: RunloopCell = {
let iconView = RunloopCell()
iconView.addOnClickListener(self, action: #selector(TCRunLoopView.pageAction))
self.scrollView.addSubview(iconView)
return iconView
}()
lazy fileprivate var rightImageView: RunloopCell = {
let iconView = RunloopCell()
self.scrollView.addSubview(iconView)
return iconView
}()
lazy fileprivate var singleImageView: RunloopCell = {
let iconView = RunloopCell()
iconView.addOnClickListener(self, action: #selector(TCRunLoopView.pageAction))
self.scrollView.addSubview(iconView)
return iconView
}()
}
// MARK:布局
extension TCRunLoopView{
override public func layoutSubviews() {
if loopDataGroup.count < 2 {
singleImageView.frame = frame
}else{
leftImageView.frame = frame
centerImageView.frame = CGRect(origin: CGPoint(x: leftImageView.frame.maxX, y: 0), size: frame.size)
rightImageView.frame = CGRect(origin: CGPoint(x: centerImageView.frame.maxX, y: 0), size: frame.size)
scrollView.bringSubview(toFront: centerImageView)
scrollView.frame = frame
pageControl.setCenterX(getCenterX())
pageControl.setY(frame.maxY - 20)
}
}
}
// MARK:UIScrollViewDelegate
extension TCRunLoopView:UIScrollViewDelegate{
@objc public func scrollViewWillBeginDragging(_ scrollView: UIScrollView) {
stop()
if delegate != nil && (delegate?.responds(to: #selector(delegate!.scrollViewWillBeginDragging(_:))))! {
delegate?.scrollViewWillBeginDragging!(scrollView)
}
}
@objc public func scrollViewDidEndDragging(_ scrollView: UIScrollView, willDecelerate decelerate: Bool) {
runloopViewFire()
if delegate != nil && (delegate?.responds(to: #selector(delegate!.scrollViewDidEndDragging(_:willDecelerate:))))! {
delegate?.scrollViewDidEndDragging!(scrollView, willDecelerate: decelerate)
}
}
@objc public func scrollViewDidScroll(_ scrollView: UIScrollView) {
loadImage(scrollView.contentOffset.x)
if delegate != nil && (delegate?.responds(to: #selector(delegate!.scrollViewDidScroll(_:))))! {
delegate?.scrollViewDidScroll!(scrollView)
}
}
fileprivate func loadImage(_ offX:CGFloat) {
if offX >= bounds.width * 2
{
currIndex = currIndex + 1
if currIndex == loopDataGroup.count - 1
{
mapImage(currIndex - 1, center: currIndex, right: 0)
}
else if currIndex == loopDataGroup.count
{
currIndex = 0
mapImage(loopDataGroup.count - 1, center: currIndex, right: currIndex+1)
}else
{
mapImage(currIndex - 1, center: currIndex, right: currIndex+1)
}
}
if offX <= 0
{
currIndex = currIndex - 1
if currIndex == 0
{
mapImage(loopDataGroup.count-1, center: 0, right: 1)
}
else if currIndex == -1
{
currIndex = loopDataGroup.count - 1
mapImage(currIndex - 1, center: currIndex, right: 0)
}else
{
mapImage(currIndex - 1, center: currIndex, right: currIndex+1)
}
}
}
func stop() {
if timer == nil { return }
if loopDataGroup.count < 2 { return }
timer!.invalidate()
timer = nil
}
func runloopViewFire() {
if loopDataGroup.count < 2 { return }
RunLoop.current.add(getTime(), forMode: RunLoopMode.defaultRunLoopMode)
}
}
extension TCRunLoopView{
fileprivate func setUpRunloopView() {
if loopDataGroup.count < 2 {
scrollView.contentSize = bounds.size
singleImageView.frame = frame
singleImageView.loopData = loopDataGroup[0]
}else{
scrollView.contentSize = CGSize(width: self.bounds.width * 3, height: 0)
runloopViewFire()
mapImage(loopDataGroup.count - 1, center: 0, right: 1)
}
}
fileprivate func mapImage(_ left:NSInteger,center:NSInteger,right:NSInteger) {
scrollView.setContentOffset(CGPoint(x: bounds.width, y: 0), animated: false)
leftImageView.loopData = loopDataGroup[left]
centerImageView.loopData = loopDataGroup[center]
rightImageView.loopData = loopDataGroup[right]
}
fileprivate func getTime()->Timer{
if (timer == nil) {
timer = Timer.scheduledTimer(timeInterval: loopInterval, target: self, selector: #selector(TCRunLoopView.timeAction), userInfo: nil, repeats: true)
}
return timer!
}
@objc func pageAction() {
delegate?.runLoopViewDidClick(self, didSelectRowAtIndex: currIndex)
// if (delegate != nil) && (delegate?.responds(to: #selector(delegate!.runLoopViewDidClick(_:didSelectRowAtIndex:)) ))!{
// delegate?.runLoopViewDidClick!(self, didSelectRowAtIndex: currIndex)
// }
}
@objc fileprivate func timeAction() {
if loopDataGroup.count < 2 { return }
scrollView.setContentOffset(CGPoint(x:scrollView.contentOffset.x + bounds.width, y: 0), animated: true)
}
}
// MARK:RunloopCell
class RunloopCell: UIView {
var loopData : LoopData?{
didSet{
iconView.sd_setImage(with: URL(string: (loopData?.imageUrl)!))
desLabel.text = loopData?.desc
}
}
lazy fileprivate var iconView: UIImageView = {
let object = UIImageView()
object.contentMode = .scaleAspectFill
self.addSubview(object)
return object
}()
lazy fileprivate var desLabel: UILabel = {
let label = UILabel()
label.font = UIFont.boldSystemFont(ofSize: 20)
label.textColor = UIColor.white
label.numberOfLines = 0
label.textAlignment = .left
label.lineBreakMode = .byCharWrapping
label.sizeToFit()
self.addSubview(label)
return label
}()
}
// MARK:布局
extension RunloopCell{
override func layoutSubviews() {
iconView.translatesAutoresizingMaskIntoConstraints = false
addConstraints(NSLayoutConstraint.constraints(withVisualFormat: "H:|[view]|", options: NSLayoutFormatOptions.alignAllLastBaseline, metrics: nil, views: ["view" : iconView]))
addConstraints(NSLayoutConstraint.constraints(withVisualFormat: "V:|[view]|", options: NSLayoutFormatOptions.alignAllLastBaseline, metrics: nil, views: ["view" : iconView]))
desLabel.translatesAutoresizingMaskIntoConstraints = false
addConstraint(NSLayoutConstraint(item: desLabel, attribute: .bottom, relatedBy: .equal, toItem: iconView, attribute: .bottom, multiplier: 1, constant: -20))
addConstraint(NSLayoutConstraint(item: desLabel, attribute: .left, relatedBy: .equal, toItem: iconView, attribute: .left, multiplier: 1, constant: 20))
addConstraint(NSLayoutConstraint(item: desLabel, attribute: .right, relatedBy: .equal, toItem: iconView, attribute: .right, multiplier: 1, constant: -20))
}
}
// MARK:添加点击事件
extension RunloopCell {
func addOnClickListener(_ target: AnyObject, action: Selector) {
let gr = UITapGestureRecognizer(target: target, action: action)
gr.numberOfTapsRequired = 1
isUserInteractionEnabled = true
addGestureRecognizer(gr)
}
}
// MARK: - 设置farme相关
fileprivate extension UIView{
func setX(_ x:CGFloat){
var frame = self.frame
frame.origin.x = x
self.frame = frame
}
func setY(_ y:CGFloat){
var frame = self.frame
frame.origin.y = y
self.frame = frame
}
func getX() ->CGFloat{
return self.frame.origin.x
}
func getY() ->CGFloat{
return self.frame.origin.y
}
func setCenterX(_ centerX:CGFloat){
var center = self.center
center.x = centerX
self.center = center
}
func setCenterY(_ centerY:CGFloat){
var center = self.center
center.y = centerY
self.center = center
}
func getCenterX() -> CGFloat{
return self.center.x
}
func getCenterY() -> CGFloat{
return self.center.y
}
func setWidth(_ width:CGFloat){
var frame = self.frame
frame.size.width = width
self.frame = frame
}
func setHeight(_ height:CGFloat){
var frame = self.frame
frame.size.height = height
self.frame = frame
}
func getHeight()->CGFloat{
return self.frame.size.height
}
func getWidth()->CGFloat{
return self.frame.size.width
}
func setSize(_ size:CGSize){
var frame = self.frame
frame.size = size
self.frame = frame
}
func getSize()->CGSize{
return self.frame.size
}
func setOrigin(_ origin:CGPoint){
var frame = self.frame
frame.origin = origin
self.frame = frame
}
func getOrigin()->CGPoint{
return self.frame.origin
}
}
public extension TCRunLoopViewDelegate{
func runLoopViewDidClick(_ loopView:TCRunLoopView, didSelectRowAtIndex index: NSInteger){}
func runLoopViewDidScroll(_ loopView: TCRunLoopView, didScrollRowAtIndex index: NSInteger){}
}
| mit | a781910b355ce2b0f5bc7ece0bbf69cb | 35.070588 | 181 | 0.625489 | 4.872467 | false | false | false | false |
yeziahehe/Gank | Gank/Views/Cells/Me/UserInfoCell.swift | 1 | 1209 | //
// UserInfoCell.swift
// Gank
//
// Created by 叶帆 on 2017/8/3.
// Copyright © 2017年 Suzhou Coryphaei Information&Technology Co., Ltd. All rights reserved.
//
import UIKit
class UserInfoCell: UITableViewCell {
@IBOutlet weak var nameLabel: UILabel!
@IBOutlet weak var loginLabel: UILabel!
@IBOutlet weak var avatarImageView: UIImageView!
override func awakeFromNib() {
super.awakeFromNib()
// Initialization code
}
func configure() {
if GankUserDefaults.isLogined {
nameLabel.text = GankUserDefaults.name.value
loginLabel.text = GankUserDefaults.login.value
avatarImageView.kf.setImage(with: URL(string: GankUserDefaults.avatarUrl.value!)!)
isUserInteractionEnabled = false
} else {
nameLabel.text = "用 GitHub 登录"
loginLabel.text = "登录后可提交干货"
avatarImageView.image = nil
isUserInteractionEnabled = true
}
}
override func setSelected(_ selected: Bool, animated: Bool) {
super.setSelected(selected, animated: animated)
// Configure the view for the selected state
}
}
| gpl-3.0 | 1f8b664e8b2c3ee1957f69ff883f353b | 27.095238 | 94 | 0.638983 | 4.836066 | false | false | false | false |
yarneo/Siren | Sample App/Sample App/AppDelegate.swift | 1 | 2006 | //
// AppDelegate.swift
// Siren
//
// Created by Arthur Sabintsev on 1/3/15.
// Copyright (c) 2015 Sabintsev iOS Projects. All rights reserved.
//
import UIKit
extension AppDelegate: SirenDelegate
{
func sirenDidShowUpdateDialog() {
print("sirenDidShowUpdateDialog")
}
func sirenUserDidCancel() {
print("sirenUserDidCancel")
}
func sirenUserDidSkipVersion() {
print("sirenUserDidSkipVersion")
}
func sirenUserDidLaunchAppStore() {
print("sirenUserDidLaunchAppStore")
exit(0)
}
}
@UIApplicationMain
class AppDelegate: UIResponder, UIApplicationDelegate {
var window: UIWindow?
func application(application: UIApplication, didFinishLaunchingWithOptions launchOptions: [NSObject: AnyObject]?) -> Bool {
window?.makeKeyAndVisible()
setupSiren()
return true
}
func setupSiren() {
let siren = Siren.sharedInstance
// Required
siren.isEnterpriseVersion = true
siren.enterpriseDownloadURL = /* enter link to plist for your enterprise app here */
siren.enterpriseVersionURL =/* enter link to json file that shows current version here */
// Optional
siren.delegate = self
// Optional
siren.debugEnabled = true;
// Optional - Defaults to .Option
// siren.alertType = .Option
// Optional - Can set differentiated Alerts for Major, Minor, Patch, and Revision Updates
siren.majorUpdateAlertType = .Option
siren.minorUpdateAlertType = .Option
siren.patchUpdateAlertType = .Option
siren.revisionUpdateAlertType = .Option
// Optional - Sets all messages to appear in Spanish. Siren supports many other languages, not just English and Spanish.
// siren.forceLanguageLocalization = .Spanish
// Required
siren.checkVersion(.Immediately)
}
}
| mit | dbb8e1e9eb7fdfac2f58a2a5d5115e60 | 25.051948 | 128 | 0.634596 | 5.421622 | false | false | false | false |
turingcorp/kitkat | kitkat/View/VGameRacket.swift | 1 | 3115 | import UIKit
class VGameRacket:UIView
{
weak var controller:CGame!
weak var layoutRacketTop:NSLayoutConstraint!
var currentCenterY:CGFloat
var expectedCenterY:CGFloat
private let kCornerRadius:CGFloat = 4
private let kWidth:CGFloat = 25
private let kHeight:CGFloat = 100
private let kLeft:CGFloat = 60
private let kDeltaY:CGFloat = 2
private var height_2:CGFloat
init(controller:CGame)
{
height_2 = kHeight / 2.0
expectedCenterY = 0
currentCenterY = 0
super.init(frame:CGRectZero)
userInteractionEnabled = false
backgroundColor = UIColor.blueColor()
clipsToBounds = true
translatesAutoresizingMaskIntoConstraints = false
layer.cornerRadius = kCornerRadius
self.controller = controller
let parent:UIView = controller.view
parent.addSubview(self)
let views:[String:AnyObject] = [
"racket":self]
let metrics:[String:AnyObject] = [
"racketLeft":kLeft,
"racketWidth":kWidth,
"racketHeight":kHeight]
parent.addConstraints(NSLayoutConstraint.constraintsWithVisualFormat(
"H:|-(racketLeft)-[racket(racketWidth)]",
options:[],
metrics:metrics,
views:views))
parent.addConstraints(NSLayoutConstraint.constraintsWithVisualFormat(
"V:[racket(racketHeight)]",
options:[],
metrics:metrics,
views:views))
let midY:CGFloat = UIScreen.mainScreen().bounds.midY
currentCenterY = midY
expectedCenterY = currentCenterY
layoutRacketTop = NSLayoutConstraint(
item:self,
attribute:NSLayoutAttribute.Top,
relatedBy:NSLayoutRelation.Equal,
toItem:parent,
attribute:NSLayoutAttribute.Top,
multiplier:1,
constant:0)
parent.addConstraint(layoutRacketTop)
updateTop()
}
required init?(coder:NSCoder)
{
fatalError()
}
private func updateTop()
{
let margin:CGFloat = currentCenterY - height_2
layoutRacketTop.constant = margin
}
//MARK: public
func touchedAt(point:CGPoint)
{
expectedCenterY = point.y
}
func tick()
{
var shouldUpdateTop:Bool = true
if currentCenterY < expectedCenterY
{
currentCenterY += kDeltaY
if currentCenterY > expectedCenterY
{
currentCenterY = expectedCenterY
}
}
else if currentCenterY > expectedCenterY
{
currentCenterY -= kDeltaY
if currentCenterY < expectedCenterY
{
currentCenterY = expectedCenterY
}
}
else
{
shouldUpdateTop = false
}
if shouldUpdateTop
{
updateTop()
}
}
} | mit | 8542f2a0be0028fcf23538bdafb6232e | 25.184874 | 77 | 0.559551 | 5.844278 | false | false | false | false |
PopovVadim/PVDSwiftAddOns | PVDSwiftAddOns/Classes/Extensions/UIKit/UILabelExtension.swift | 1 | 976 | //
// UILabel.swift
// Pods-PVDSwiftAddOns_Tests
//
// Created by Вадим Попов on 10/24/17.
//
import Foundation
import UIKit
/**
*
*
*/
public extension UILabel {
/**
*/
func blinkText(withColor color: UIColor, duration: Double = 0.5) {
let initialColor = self.textColor
UIView.transition(with: self, duration: duration/2, options: .transitionCrossDissolve, animations: {
self.textColor = color
}, completion: { _ in
UIView.transition(with: self, duration: duration/2, options: .transitionCrossDissolve, animations: {
self.textColor = initialColor
}, completion: nil)
})
}
/**
*/
func apply (_ textDescriptor: TextDescriptor) {
self.textColor = textDescriptor.color
self.textAlignment = textDescriptor.alignment
self.font = textDescriptor.font
self.numberOfLines = textDescriptor.numberOfLines
}
}
| mit | e359b56264c96b49bc882993666f1a79 | 24.421053 | 112 | 0.619048 | 4.410959 | false | false | false | false |
hgani/ganilib-ios | glib/Classes/View/Layout/GScrollPanel.swift | 1 | 2446 | import UIKit
open class GScrollPanel: UIScrollView, IContainer {
private var helper: ViewHelper!
private let contentView = GVerticalPanel()
public var size: CGSize {
return helper.size
}
public init() {
super.init(frame: .zero)
helper = ViewHelper(self)
// See https://github.com/zaxonus/AutoLayScroll
// self.translatesAutoresizingMaskIntoConstraints = false
contentView.translatesAutoresizingMaskIntoConstraints = false
addSubview(contentView)
contentView.snp.makeConstraints { make in
make.centerX.equalTo(self)
make.width.equalTo(self)
make.top.equalTo(self)
make.bottom.equalTo(self)
}
}
public required init?(coder _: NSCoder) {
fatalError("Not supported")
}
open override func didMoveToSuperview() {
super.didMoveToSuperview()
helper.didMoveToSuperview()
}
public func width(_ width: Int) -> Self {
helper.width(width)
return self
}
public func width(_ width: LayoutSize) -> Self {
helper.width(width)
return self
}
public func width(weight: Float) -> Self {
helper.width(weight: weight)
return self
}
public func height(_ height: Int) -> Self {
helper.height(height)
return self
}
public func height(_ height: LayoutSize) -> Self {
helper.height(height)
return self
}
public func clearViews() {
contentView.clearViews()
}
public func addView(_ view: UIView, top: Float = 0) {
contentView.addView(view, top: top)
}
public func append(_ view: UIView, top: Float = 0) -> Self {
_ = contentView.append(view, top: top)
return self
}
public func paddings(top: Float? = nil, left: Float? = nil, bottom: Float? = nil, right: Float? = nil) -> Self {
_ = contentView.paddings(top: top, left: left, bottom: bottom, right: right)
return self
}
// UIScrollView delays touch event handling by default.
public func delayTouch(_ delay: Bool) -> Self {
delaysContentTouches = delay
return self
}
public func color(bg: UIColor) -> Self {
backgroundColor = bg
return self
}
public func done() {
// End chaining
}
// public func test() {
// contentView.height(300)
// }
}
| mit | 522b01e022d5b958fe706ca42f26dc2e | 23.46 | 116 | 0.59444 | 4.512915 | false | false | false | false |
TrustWallet/trust-wallet-ios | Trust/Transactions/Coordinators/TransactionsCoordinator.swift | 1 | 1101 | // Copyright DApps Platform Inc. All rights reserved.
import Foundation
import UIKit
//
//class TransactionsCoordinator: RootCoordinator {
//
// let session: WalletSession
// let storage: TransactionsStorage
// let network: NetworkProtocol
// var coordinators: [Coordinator] = []
//
// var rootViewController: UIViewController {
// return transactionViewController
// }
//
// lazy var viewModel: TransactionsViewModel = {
// return TransactionsViewModel(
// network: network,
// storage: storage,
// session: session
// )
// }()
//
// lazy var transactionViewController: TransactionsViewController = {
// let controller = TransactionsViewController(
// session: session,
// viewModel: viewModel
// )
// return controller
// }()
//
// init(
// session: WalletSession,
// storage: TransactionsStorage,
// network: NetworkProtocol
// ) {
// self.session = session
// self.storage = storage
// self.network = network
// }
//}
| gpl-3.0 | b1620e4ea58cd1bcaaa1f59dac543079 | 25.214286 | 72 | 0.599455 | 4.421687 | false | false | false | false |
sebastiangrail/Mars-Orbiter | MarsOrbiter/Distance.swift | 1 | 2430 | //
// Distance.swift
// MarsOrbiter
//
// Created by Sebastian Grail on 9/11/2015.
// Copyright © 2015 Sebastian Grail. All rights reserved.
//
// Mark: Metric
public typealias Meters = Unit<MeterTrait>
public typealias Meter = Meters
public struct MeterTrait: UnitTrait {
public typealias BaseTrait = MeterTrait
public static func toBaseUnit () -> Double {
return 1
}
public static func abbreviation() -> String {
return "m"
}
}
public typealias Millimeters = Unit<MillimeterTrait>
public typealias Millimeter = Millimeters
public struct MillimeterTrait: UnitTrait {
public typealias BaseTrait = MeterTrait
public static func toBaseUnit () -> Double {
return 1/1000
}
public static func abbreviation() -> String {
return "mm"
}
}
public typealias Centimeters = Unit<CentimetersTrait>
public typealias Centimeter = Centimeters
public struct CentimetersTrait: UnitTrait {
public typealias BaseTrait = MeterTrait
public static func toBaseUnit () -> Double {
return 1/100
}
public static func abbreviation() -> String {
return "cm"
}
}
public typealias Kilometers = Unit<KilometerTrait>
public typealias Kilometer = Kilometers
public struct KilometerTrait: UnitTrait {
public typealias BaseTrait = MeterTrait
public static func toBaseUnit () -> Double {
return 1000
}
public static func abbreviation() -> String {
return "km"
}
}
// Mark: Imperial
public typealias Inches = Unit<InchTrait>
public typealias Inch = Inches
public struct InchTrait: UnitTrait {
public typealias BaseTrait = MeterTrait
public static func toBaseUnit () -> Double {
return 0.0254
}
public static func abbreviation() -> String {
return "in"
}
}
public typealias Feet = Unit<FootTrait>
public typealias Foot = Feet
public struct FootTrait: UnitTrait {
public typealias BaseTrait = MeterTrait
public static func toBaseUnit () -> Double {
return 0.3048
}
public static func abbreviation() -> String {
return "ft"
}
}
public typealias Miles = Unit<MileTrait>
public typealias Mile = Miles
public struct MileTrait: UnitTrait {
public typealias BaseTrait = MeterTrait
public static func toBaseUnit () -> Double {
return 1609.344
}
public static func abbreviation() -> String {
return "miles"
}
}
| mit | 077659b53706ce0eb37823f12c600e32 | 24.302083 | 58 | 0.680939 | 4.523277 | false | false | false | false |
izeni-team/retrolux | Retrolux/Query.swift | 1 | 1207 | //
// Query.swift
// Retrolux
//
// Created by Bryan Henderson on 1/26/17.
// Copyright © 2017 Bryan. All rights reserved.
//
import Foundation
public struct Query: SelfApplyingArg {
public let value: String
public init(_ nameOrValue: String) {
self.value = nameOrValue
}
public static func apply(arg: BuilderArg, to request: inout URLRequest) {
if let creation = arg.creation as? Query, let starting = arg.starting as? Query {
guard let url = request.url else {
return
}
guard var components = URLComponents(url: url, resolvingAgainstBaseURL: false) else {
return
}
let name = creation.value
let value = starting.value
var queryItems = components.queryItems ?? []
queryItems.append(URLQueryItem(name: name, value: value))
components.queryItems = queryItems
if let newUrl = components.url {
request.url = newUrl
} else {
print("Error: Failed to apply query `\(name)=\(value)`")
}
}
}
}
| mit | 8d8533260e4b0f6fa71079fc8dcb4928 | 27.714286 | 97 | 0.541459 | 5.004149 | false | false | false | false |
kirby10023/DemoShowcaseProject | SampleProject/Helpers/ActivityViewPresentor.swift | 1 | 1398 | //
// ActivityViewPresentor.swift
// SampleProject
//
// Created by Kirby on 6/20/17.
// Copyright © 2017 Kirby. All rights reserved.
//
import UIKit
/// Used for view controllers that need to present an activity indicator
protocol ActivityIndicatorPresentor {
var activityIndicator: ActivityProgressHud { get }
func showActivityIndicator(title: String)
func hideActivityIndicator()
}
extension ActivityIndicatorPresentor where Self: UIViewController {
func showActivityIndicator(title: String) {
DispatchQueue.main.async {
// prevents another progress hud from appearing if it already exists
if self.isActivityIndicatorPresent {
return
}
self.activityIndicator.text = title
self.activityIndicator.frame = CGRect(x: 0, y: 0, width: 85, height: 85)
self.activityIndicator.center = CGPoint(x: self.view.bounds.size.width / 2, y: self.view.bounds.height / 2)
self.view.addSubview(self.activityIndicator)
}
}
func hideActivityIndicator() {
DispatchQueue.main.async {
self.activityIndicator.removeFromSuperview()
}
}
/// Checks to see if a activity indicator is already presented in the view
private var isActivityIndicatorPresent: Bool {
let allActivityIndicators = view.subviews.compactMap { $0 as? ActivityProgressHud }
return (allActivityIndicators.count >= 1 ? true : false)
}
}
| mit | fa1b06eda6be022a76c14bb26eebc044 | 25.865385 | 113 | 0.722262 | 4.550489 | false | false | false | false |
amotz/walkingintherhythm | walkingintherhythm/StepsViewController.swift | 1 | 2210 | //
// StepsViewController.swift
// walkingintherhythm
//
// Created by amotz on 2016/06/29.
// Copyright © 2016年 amotz. All rights reserved.
//
import UIKit
import CoreMotion
class StepsViewController: UIViewController {
// MARK: Properties
@IBOutlet weak var StepsLabel: UILabel!
@IBOutlet weak var LeftLabel: UILabel!
@IBOutlet weak var AchieveLabel: UILabel!
@objc let userDefaults: UserDefaults = UserDefaults(suiteName: Const().suiteName)!
@objc var myPedometer: CMPedometer!
override func viewWillAppear(_ animated: Bool) {
self.navigationController?.setNavigationBarHidden(true, animated: false)
self.AchieveLabel.isHidden = true
self.userDefaults.register(defaults: ["goalStepsaDay": 4000])
let goalStepsaDay: Int = self.userDefaults.object(forKey: "goalStepsaDay") as! Int
self.LeftLabel.text = "\(goalStepsaDay)"
let startOfToday: Date = Calendar(identifier: .gregorian).startOfDay(for: Date())
countSteps(from: startOfToday)
}
@objc func countSteps(from: Date) {
let goalStepsaDay: Int = Int(self.LeftLabel.text!)!
myPedometer = CMPedometer()
myPedometer.startUpdates(from: from, withHandler: { (data, error) -> Void in
if error==nil {
DispatchQueue.main.async(execute: {
if data != nil && error == nil {
let todaysSteps: Int = Int(truncating: data!.numberOfSteps)
self.StepsLabel.text = "\(todaysSteps)"
var stepsToGo: Int
if goalStepsaDay <= todaysSteps {
stepsToGo = 0
} else {
stepsToGo = goalStepsaDay - todaysSteps
}
self.LeftLabel.text = "\(stepsToGo)"
if stepsToGo==0 {
self.AchieveLabel.isHidden = false
}
}
})
}
})
}
}
| mit | 81e67fbdb0b7c75b04587533d27a5a72 | 32.953846 | 90 | 0.529225 | 5.180751 | false | false | false | false |
mitchtreece/Bulletin | Bulletin/Classes/UIApplication+Bulletin.swift | 1 | 1447 | //
// UIApplication+Bulletin.swift
// Bulletin
//
// Created by Mitch Treece on 7/17/17.
// Copyright © 2017 Mitch Treece. All rights reserved.
//
import UIKit
internal typealias UIStatusBarAppearance = (style: UIStatusBarStyle, hidden: Bool)
internal extension UIApplication {
var currentStatusBarAppearance: UIStatusBarAppearance {
guard let rootVC = keyWindow?.rootViewController else { return (.default, false) }
if let nav = rootVC as? UINavigationController {
let style = nav.topViewController?.preferredStatusBarStyle ?? .default
let hidden = nav.topViewController?.prefersStatusBarHidden ?? false
return (style, hidden)
}
else if let tab = rootVC as? UITabBarController {
let style = tab.selectedViewController?.preferredStatusBarStyle ?? .default
let hidden = tab.selectedViewController?.prefersStatusBarHidden ?? false
return (style, hidden)
}
return (rootVC.preferredStatusBarStyle, rootVC.prefersStatusBarHidden)
}
var currentKeyboardWindow: UIWindow? {
for window in UIApplication.shared.windows {
if NSStringFromClass(window.classForCoder) == "UIRemoteKeyboardWindow" {
return window
}
}
return nil
}
}
| mit | 6a1cfbb3e78d24b030b2f0d8b9b4800e | 27.92 | 90 | 0.612725 | 6.050209 | false | false | false | false |
yangyu92/YYProgressAnimation | YYProgressAnimation/YYGradualProgressView.swift | 1 | 8165 | //
// YYGradualProgressView.swift
// XFT315
//
// Created by canyou on 16/9/3.
// Copyright © 2016年 canyou. All rights reserved.
//
import UIKit
class YYGradualProgressView: UIView,CAAnimationDelegate{
var viewWidth: CGFloat = 0
var maskLayuer: CAGradientLayer?
var progresst: CGFloat? = 0.0
var colorArray: Array <AnyObject>?
override var frame: CGRect {
willSet {
if frame.size.width == viewWidth {
self.isHidden = true
}
super.frame = frame
var maskRect = frame
maskRect.origin.x = 0
maskRect.origin.y = 0
maskRect.size.width = self.bounds.width * progresst! + 0
maskLayuer?.frame = maskRect
}
}
override init(frame: CGRect) {
super.init(frame: frame)
//创建CAGradientLayer实例并设置参数
maskLayuer = turquoiseColor()
//设置其frame以及插入view的layer
var maskRect = frame
maskRect.origin.x = 0
maskRect.origin.y = 0
maskRect.size.width = self.bounds.width * progresst! + 0
maskLayuer!.frame = maskRect
self.isUserInteractionEnabled = false
self.autoresizingMask = UIViewAutoresizing.flexibleWidth
// self.layer.addSublayer(maskLayuer!)
self.layer.insertSublayer(maskLayuer!, at: 0)
gradinetAnimate()
}
func turquoiseColor() -> CAGradientLayer {
let topColor = UIColor.red
let bottomColor = UIColor.white
colorArray = [topColor.cgColor, bottomColor.cgColor,topColor.cgColor]
let gradientLocations: [CGFloat] = [0.0, 0.0001,0.0002]
let gradientLayer: CAGradientLayer = CAGradientLayer()
gradientLayer.startPoint = CGPoint(x: 0.0, y: 0.0)
gradientLayer.endPoint = CGPoint(x: 1.0, y: self.frame.size.height/(self.frame.size.width + 0))
gradientLayer.colors = colorArray
gradientLayer.locations = gradientLocations as [NSNumber]?
return gradientLayer
}
func setProgress(_ value: CGFloat) {
if progresst != value {
progresst = min(1.0, fabs(value))
}
maskLayuer?.frame = setMaskRect(value)
}
func setMaskRect(_ progress: CGFloat) -> CGRect {
var maskRect = self.maskLayuer?.frame
maskRect!.size.width = (self.frame.size.width * progress)*2 + 0
return maskRect!
}
func gradinetAnimate() {
let gradient = CABasicAnimation(keyPath:"locations")
gradient.fromValue = [-0.02,0,0.02]
gradient.toValue = [0.98,1,1.02]
gradient.duration = 4
gradient.repeatCount = HUGE
gradient.setValue("gradient", forKey: "gradient")
// maskLayuer?.type = kCAGradientLayerAxial 像素均匀
maskLayuer?.add(gradient, forKey: "colors")
}
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
func start() {
maskLayuer!.isHidden = false
maskLayuer!.removeAllAnimations()
gradinetAnimate()
let animation = CABasicAnimation(keyPath: "bounds")
animation.fromValue = NSValue(cgRect: setMaskRect(0.0))
animation.toValue = NSValue(cgRect: setMaskRect(0.7))
// animation.byValue = NSValue(CGRect: self.bounds)
animation.duration = 3.0
animation.timingFunction = CAMediaTimingFunction(name: kCAMediaTimingFunctionEaseOut)
animation.repeatCount = 1
animation.isRemovedOnCompletion = false
animation.delegate = self
animation.fillMode = kCAFillModeForwards
animation.autoreverses = false // 执行逆动画
animation.setValue("animation1", forKey: "animation1")
maskLayuer?.add(animation, forKey: "animation")
let animation2 = CABasicAnimation(keyPath: "bounds")
animation2.toValue = NSValue(cgRect: setMaskRect(0.9))
// animation2.byValue = NSValue(CGRect: self.bounds)
animation2.beginTime = CACurrentMediaTime() + animation.duration + animation.beginTime + 3.8
animation2.duration = 2.0
animation2.timingFunction = CAMediaTimingFunction(name: kCAMediaTimingFunctionLinear)
animation2.repeatCount = 1
// animation.additive = true
animation2.isRemovedOnCompletion = false
animation2.delegate = self
animation2.fillMode = kCAFillModeForwards
animation2.autoreverses = false // 执行逆动画
animation2.setValue("animation2", forKey: "animation2")
maskLayuer?.add(animation2, forKey: "animation2")
}
func animationDidStop(_ anim: CAAnimation, finished flag: Bool) {
if (((anim.value(forKey: "animation1") as AnyObject).isEqual("animation1"))) {
if flag {
// print("动画1完成\(self.maskLayuer!.modelLayer().bounds.size.width)")
print("动画1完成(正确结束)\(self.maskLayuer!.presentation()!.bounds.size.width)")
}else {
print("动画1完成(错误结束)\(maskLayuer!.bounds.size.width)")
maskLayuer?.removeAnimation(forKey: "animation3")
maskLayuer?.removeAnimation(forKey: "opacity1")
}
}
if (((anim.value(forKey: "animation2") as AnyObject).isEqual("animation2"))) {
if flag {
// print("动画1完成\(self.maskLayuer!.modelLayer().bounds.size.width)")
print("动画2完成(正确结束)")
}
else {
maskLayuer?.removeAnimation(forKey: "animation3")
maskLayuer?.removeAnimation(forKey: "opacity1")
print("动画2完成(错误结束)")
}
}
if (((anim.value(forKey: "animation3") as AnyObject).isEqual("animation3"))) {
// maskLayuer?.removeAnimationForKey("colors")
// maskLayuer?.removeAnimationForKey("animation")
// maskLayuer?.removeAnimationForKey("animation2")
// maskLayuer?.removeAnimationForKey("animation3")
// maskLayuer?.removeAnimationForKey("opacity1")
if flag {
maskLayuer!.removeAllAnimations()
maskLayuer?.isHidden = true
print("动画3完成(正确结束)")
}
else {
maskLayuer?.removeAnimation(forKey: "opacity1")
print("动画3完成(错误结束)")
}
}
}
func stop() {
maskLayuer?.removeAnimation(forKey: "colors")
let animation3 = CABasicAnimation(keyPath: "bounds")
animation3.toValue = NSValue(cgRect: setMaskRect(1))
animation3.duration = 0.3
animation3.timingFunction = CAMediaTimingFunction(name: kCAMediaTimingFunctionLinear)
animation3.repeatCount = 1.0
animation3.isRemovedOnCompletion = false
animation3.delegate = self
animation3.fillMode = kCAFillModeForwards
animation3.autoreverses = false // 执行逆动画
animation3.setValue("animation3", forKey: "animation3")
maskLayuer?.add(animation3, forKey: "animation3")
let opaqueAnimate = CABasicAnimation(keyPath: "opacity")
opaqueAnimate.fromValue = 1.0
opaqueAnimate.toValue = 0.0
opaqueAnimate.repeatCount = 1
opaqueAnimate.duration = 0.5
opaqueAnimate.isRemovedOnCompletion = false
opaqueAnimate.delegate = self
opaqueAnimate.fillMode = kCAFillModeForwards
opaqueAnimate.autoreverses = false // 执行逆动画
maskLayuer?.add(opaqueAnimate, forKey: "opacity1")
//// let caa = maskLayuer?.animationForKey("animation")
// maskLayuer!.removeAllAnimations()
//// maskLayuer?.removeAnimationForKey("animation")
//// maskLayuer?.removeAnimationForKey("animation2")
}
}
| mit | ca6c9ef64bcaa52ba20a53b3953a10a5 | 36.233645 | 103 | 0.601782 | 4.933746 | false | false | false | false |
meninsilicium/apple-swifty | DispatchBlock.swift | 1 | 781 | //
// author: fabrice truillot de chambrier
// created: 23.03.2015
//
// license: see license.txt
//
// © 2015-2015, men in silicium sàrl
//
import Foundation
import Dispatch
public class DispatchBlock {
public var function: (() -> Void)?
public var queue: DispatchQueue?
private var dispatched: Bool = false
private var executing: Bool = false
public init( function: () -> Void ) {
self.function = function
self.queue = nil
}
private func async() -> Bool {
if self.dispatched { return true }
if let queue = self.queue, let function = self.function {
self.dispatched = true
queue.async {
self.executing = true
function()
self.executing = false
self.function = nil
}
return true
}
return false
}
}
| mpl-2.0 | 8993e365ca3816d89915db99c5b68d1d | 15.574468 | 59 | 0.641849 | 3.343348 | false | false | false | false |
KinoAndWorld/DailyMood | DailyMood/Controller/Conponent/AddDailyComponent.swift | 1 | 4169 | //
// AddDailyComponent.swift
// DailyMood
//
// Created by kino on 14-7-14.
// Copyright (c) 2014 kino. All rights reserved.
//
import Foundation
@objc(AddDailyComponent)
class AddDailyComponent: NSObject {
weak var hostController:UIViewController?
init(controller:UIViewController){
hostController = controller
}
func showComponent(){
}
}
class MoodComponent : AddDailyComponent{
override func showComponent() {
}
}
class PictureComponent : AddDailyComponent ,
UIImagePickerControllerDelegate,
UINavigationControllerDelegate,
GKImagePickerDelegate{
var pictrueFinishBlock:(image:UIImage)->Void?
var isExistPicture:Bool = false
init(controller: UIViewController, pictureFinish:(image:UIImage)->Void){
pictrueFinishBlock = pictureFinish
super.init(controller: controller)
}
deinit{
println("opps!!!")
}
override func showComponent() {
var sheet = DoActionSheet()
sheet.nAnimationType = 2 //todo i don not how to use the objc's enum in swift...
//DoAlertViewTransitionStyle.DoASTransitionStylePop doesn't work
sheet.showC("Chose a mood for now :)",
cancel: "Cancel",
buttons: ["take picture","picture library"],
result: {[weak self] result in
println("result : \(result)")
// self.takePhoto()
if let weakSelf = self{
switch result{
case 0:
weakSelf.takePhoto()
println("take photo")
case 1:
weakSelf.selectPhotoFromLib()
default:
println("opps?")
}
}
})
}
func takePhoto(){
if(UIImagePickerController.isSourceTypeAvailable(UIImagePickerControllerSourceType.Camera)){
// var picker:UIImagePickerController = UIImagePickerController()
// picker.delegate = self
// picker.allowsEditing = true
// picker.sourceType = UIImagePickerControllerSourceType.Camera
// hostController?.presentViewController(picker, animated: true, completion: nil)
imagePicker = GKImagePicker(sourceType: UIImagePickerControllerSourceType.Camera)
imagePicker.cropSize = CGSizeMake(320, 160);
imagePicker.delegate = self;
imagePicker.resizeableCropArea = false;
hostController?.presentViewController(imagePicker.imagePickerController, animated: true, completion: nil)
}
}
var imagePicker = GKImagePicker(sourceType: UIImagePickerControllerSourceType.PhotoLibrary)
func selectPhotoFromLib(){
imagePicker = GKImagePicker(sourceType: UIImagePickerControllerSourceType.PhotoLibrary)
imagePicker.cropSize = CGSizeMake(320, 160);
imagePicker.delegate = self;
imagePicker.resizeableCropArea = false;
println("\(hostController) and \(imagePicker.imagePickerController)")
hostController?.presentViewController(imagePicker.imagePickerController, animated: true, completion: nil)
}
///UIImagePickerControllerDelegate
func imagePicker(imagePicker: GKImagePicker!, pickedImage image: UIImage!) {
// var chosenImage = info[UIImagePickerControllerEditedImage] as UIImage
imagePicker.imagePickerController.dismissViewControllerAnimated(true, completion:nil)
pictrueFinishBlock(image: image)?
}
func imagePickerDidCancel(imagePicker: GKImagePicker!) {
imagePicker.imagePickerController.dismissViewControllerAnimated(true, completion:nil)
}
}
class LocationComponent : AddDailyComponent{
override func showComponent() {
}
}
class WeatherComponent : AddDailyComponent{
override func showComponent() {
}
} | mit | ca626a816328bca9ea4731ef14560937 | 30.119403 | 117 | 0.611657 | 6.103953 | false | false | false | false |
fgengine/quickly | Quickly/Views/Fields/Text/QTextField.swift | 1 | 34034 | //
// Quickly
//
public protocol IQTextFieldSuggestion : class {
func autoComplete(_ text: String) -> String?
func variants(_ text: String) -> [String]
}
public protocol IQTextFieldSuggestionController : IQCollectionController {
typealias SelectSuggestionClosure = (_ controller: IQTextFieldSuggestionController, _ suggestion: String) -> Void
var onSelectSuggestion: SelectSuggestionClosure? { set get }
func set(variants: [String])
}
public protocol IQTextFieldObserver : class {
func beginEditing(textField: QTextField)
func editing(textField: QTextField)
func endEditing(textField: QTextField)
func pressed(textField: QTextField, action: QFieldAction)
func pressedClear(textField: QTextField)
func pressedReturn(textField: QTextField)
func select(textField: QTextField, suggestion: String)
}
open class QTextFieldStyleSheet : QDisplayStyleSheet {
public var requireValidator: Bool
public var validator: IQStringValidator?
public var form: IQFieldForm?
public var formatter: IQStringFormatter?
public var textInsets: UIEdgeInsets
public var textStyle: IQTextStyle?
public var editingInsets: UIEdgeInsets?
public var placeholderInsets: UIEdgeInsets?
public var placeholder: IQText?
public var clearButtonMode: UITextField.ViewMode
public var typingStyle: IQTextStyle?
public var autocapitalizationType: UITextAutocapitalizationType
public var autocorrectionType: UITextAutocorrectionType
public var spellCheckingType: UITextSpellCheckingType
public var keyboardType: UIKeyboardType
public var keyboardAppearance: UIKeyboardAppearance
public var returnKeyType: UIReturnKeyType
public var enablesReturnKeyAutomatically: Bool
public var isSecureTextEntry: Bool
public var textContentType: UITextContentType!
public var isEnabled: Bool
public var toolbarStyle: QToolbarStyleSheet?
public var toolbarActions: QFieldAction
public var suggestion: IQTextFieldSuggestion?
public var suggestionStyle: QCollectionStyleSheet?
public var suggestionController: IQTextFieldSuggestionController?
public init(
requireValidator: Bool = false,
validator: IQStringValidator? = nil,
form: IQFieldForm? = nil,
formatter: IQStringFormatter? = nil,
textInsets: UIEdgeInsets = UIEdgeInsets.zero,
textStyle: IQTextStyle? = nil,
editingInsets: UIEdgeInsets? = nil,
placeholderInsets: UIEdgeInsets? = nil,
placeholder: IQText? = nil,
clearButtonMode: UITextField.ViewMode = .never,
typingStyle: IQTextStyle? = nil,
autocapitalizationType: UITextAutocapitalizationType = .none,
autocorrectionType: UITextAutocorrectionType = .default,
spellCheckingType: UITextSpellCheckingType = .default,
keyboardType: UIKeyboardType = .default,
keyboardAppearance: UIKeyboardAppearance = .default,
returnKeyType: UIReturnKeyType = .default,
enablesReturnKeyAutomatically: Bool = true,
isSecureTextEntry: Bool = false,
textContentType: UITextContentType! = nil,
isEnabled: Bool = true,
toolbarStyle: QToolbarStyleSheet? = nil,
toolbarActions: QFieldAction = [],
suggestion: IQTextFieldSuggestion? = nil,
suggestionStyle: QCollectionStyleSheet? = nil,
suggestionController: IQTextFieldSuggestionController? = nil,
backgroundColor: UIColor? = nil,
tintColor: UIColor? = nil,
cornerRadius: QViewCornerRadius = .none,
border: QViewBorder = .none,
shadow: QViewShadow? = nil
) {
self.requireValidator = requireValidator
self.validator = validator
self.form = form
self.formatter = formatter
self.textInsets = textInsets
self.textStyle = textStyle
self.editingInsets = editingInsets
self.placeholderInsets = placeholderInsets
self.placeholder = placeholder
self.clearButtonMode = clearButtonMode
self.autocapitalizationType = autocapitalizationType
self.autocorrectionType = autocorrectionType
self.spellCheckingType = spellCheckingType
self.keyboardType = keyboardType
self.keyboardAppearance = keyboardAppearance
self.returnKeyType = returnKeyType
self.enablesReturnKeyAutomatically = enablesReturnKeyAutomatically
self.isSecureTextEntry = isSecureTextEntry
self.textContentType = textContentType
self.isEnabled = isEnabled
self.toolbarStyle = toolbarStyle
self.toolbarActions = toolbarActions
self.suggestion = suggestion
self.suggestionStyle = suggestionStyle
self.suggestionController = suggestionController
super.init(
backgroundColor: backgroundColor,
tintColor: tintColor,
cornerRadius: cornerRadius,
border: border,
shadow: shadow
)
}
public init(_ styleSheet: QTextFieldStyleSheet) {
self.requireValidator = styleSheet.requireValidator
self.validator = styleSheet.validator
self.form = styleSheet.form
self.formatter = styleSheet.formatter
self.textInsets = styleSheet.textInsets
self.textStyle = styleSheet.textStyle
self.editingInsets = styleSheet.editingInsets
self.placeholderInsets = styleSheet.placeholderInsets
self.placeholder = styleSheet.placeholder
self.clearButtonMode = styleSheet.clearButtonMode
self.autocapitalizationType = styleSheet.autocapitalizationType
self.autocorrectionType = styleSheet.autocorrectionType
self.spellCheckingType = styleSheet.spellCheckingType
self.keyboardType = styleSheet.keyboardType
self.keyboardAppearance = styleSheet.keyboardAppearance
self.returnKeyType = styleSheet.returnKeyType
self.enablesReturnKeyAutomatically = styleSheet.enablesReturnKeyAutomatically
self.isSecureTextEntry = styleSheet.isSecureTextEntry
self.textContentType = styleSheet.textContentType
self.isEnabled = styleSheet.isEnabled
self.toolbarStyle = styleSheet.toolbarStyle
self.toolbarActions = styleSheet.toolbarActions
self.suggestion = styleSheet.suggestion
self.suggestionStyle = styleSheet.suggestionStyle
self.suggestionController = styleSheet.suggestionController
super.init(styleSheet)
}
}
public class QTextField : QDisplayView, IQField {
public typealias ShouldClosure = (_ textField: QTextField) -> Bool
public typealias Closure = (_ textField: QTextField) -> Void
public typealias ActionClosure = (_ textField: QTextField, _ action: QFieldAction) -> Void
public typealias SelectSuggestionClosure = (_ textField: QTextField, _ suggestion: String) -> Void
public var requireValidator: Bool = false
public var validator: IQStringValidator? {
willSet { self._field.delegate = nil }
didSet {self._field.delegate = self._fieldDelegate }
}
public var form: IQFieldForm? {
didSet(oldValue) {
if self.form !== oldValue {
if let form = oldValue {
form.remove(field: self)
}
if let form = self.form {
form.add(field: self)
}
}
}
}
public var formatter: IQStringFormatter? {
willSet {
if let formatter = self.formatter {
if let text = self._field.text {
var caret: Int
if let selected = self._field.selectedTextRange {
caret = self._field.offset(from: self._field.beginningOfDocument, to: selected.end)
} else {
caret = text.count
}
self._field.text = formatter.unformat(text, caret: &caret)
if let position = self._field.position(from: self._field.beginningOfDocument, offset: caret) {
self._field.selectedTextRange = self._field.textRange(from: position, to: position)
}
}
}
}
didSet {
if let formatter = self.formatter {
if let text = self._field.text {
var caret: Int
if let selected = self._field.selectedTextRange {
caret = self._field.offset(from: self._field.beginningOfDocument, to: selected.end)
} else {
caret = text.count
}
self._field.text = formatter.format(text, caret: &caret)
if let position = self._field.position(from: self._field.beginningOfDocument, offset: caret) {
self._field.selectedTextRange = self._field.textRange(from: position, to: position)
}
}
}
if let form = self.form {
form.validation()
}
}
}
public var textInsets: UIEdgeInsets {
set(value) { self._field.textInsets = value }
get { return self._field.textInsets }
}
public var textStyle: IQTextStyle? {
didSet {
var attributes: [NSAttributedString.Key: Any] = [:]
if let textStyle = self.textStyle {
attributes = textStyle.attributes
self._field.font = attributes[.font] as? UIFont
self._field.textColor = attributes[.foregroundColor] as? UIColor
if let paragraphStyle = attributes[.paragraphStyle] as? NSParagraphStyle {
self._field.textAlignment = paragraphStyle.alignment
} else {
self._field.textAlignment = .left
}
} else {
if let font = self._field.font {
attributes[.font] = font
}
if let textColor = self._field.textColor {
attributes[.foregroundColor] = textColor
}
let paragraphStyle = NSMutableParagraphStyle()
paragraphStyle.alignment = self._field.textAlignment
attributes[.paragraphStyle] = paragraphStyle
}
self._field.defaultTextAttributes = Dictionary(uniqueKeysWithValues:
attributes.lazy.map { (NSAttributedString.Key(rawValue: $0.key.rawValue), $0.value) }
)
}
}
public var text: String {
set(value) {
self._field.text = value
if let form = self.form {
form.validation()
}
}
get {
if let text = self._field.text {
return text
}
return ""
}
}
public var unformatText: String {
set(value) {
if let formatter = self.formatter {
var caret: Int
if let selected = self._field.selectedTextRange {
caret = self._field.offset(from: self._field.beginningOfDocument, to: selected.end)
} else {
caret = text.count
}
self._field.text = formatter.format(value, caret: &caret)
if let position = self._field.position(from: self._field.beginningOfDocument, offset: caret) {
self._field.selectedTextRange = self._field.textRange(from: position, to: position)
}
} else {
self._field.text = value
}
if let form = self.form {
form.validation()
}
}
get {
var result: String
if let text = self._field.text {
if let formatter = self.formatter {
result = formatter.unformat(text)
} else {
result = text
}
} else {
result = ""
}
return result
}
}
public var editingInsets: UIEdgeInsets? {
set(value) { self._field.editingInsets = value }
get { return self._field.editingInsets }
}
public var placeholderInsets: UIEdgeInsets? {
set(value) { self._field.placeholderInsets = value }
get { return self._field.placeholderInsets }
}
public var typingStyle: IQTextStyle? {
didSet {
if let typingStyle = self.typingStyle {
self._field.allowsEditingTextAttributes = true
self._field.typingAttributes = Dictionary(uniqueKeysWithValues:
typingStyle.attributes.lazy.map { (NSAttributedString.Key(rawValue: $0.key.rawValue), $0.value) }
)
} else {
self._field.allowsEditingTextAttributes = false
self._field.typingAttributes = nil
}
}
}
public var isValid: Bool {
get {
guard let validator = self.validator else { return true }
return validator.validate(self.unformatText).isValid
}
}
public var placeholder: IQText? {
set(value) {
if let text = value {
if let attributed = text.attributed {
self._field.attributedPlaceholder = attributed
} else if let font = text.font, let color = text.color {
self._field.attributedPlaceholder = NSAttributedString(string: text.string, attributes: [
.font: font,
.foregroundColor: color
])
} else {
self._field.attributedPlaceholder = nil
}
} else {
self._field.attributedPlaceholder = nil
}
}
get {
if let attributed = self._field.attributedPlaceholder {
return QAttributedText(attributed: attributed)
}
return nil
}
}
public var clearButtonMode: UITextField.ViewMode {
set(value) { self._field.clearButtonMode = value }
get { return self._field.clearButtonMode }
}
public var isEnabled: Bool {
set(value) { self._field.isEnabled = value }
get { return self._field.isEnabled }
}
public var isEditing: Bool {
get { return self._field.isEditing }
}
public lazy var toolbar: QToolbar = QToolbar(items: self._toolbarItems())
public var toolbarActions: QFieldAction = [] {
didSet(oldValue) {
if self.toolbarActions != oldValue {
let items = self._toolbarItems()
self.toolbar.items = items
self.toolbar.isHidden = self.toolbarActions.isEmpty
self._updateAccessoryView()
}
}
}
public var suggestion: IQTextFieldSuggestion? {
didSet(oldValue) {
if self.suggestion !== oldValue {
self._updateAccessoryView()
}
}
}
public lazy var suggestionView: QCollectionView = {
let layout = UICollectionViewFlowLayout()
layout.scrollDirection = .horizontal
let view = QCollectionView(frame: CGRect(x: 0, y: 0, width: UIScreen.main.bounds.width, height: 50), collectionViewLayout: layout)
return view
}()
public var suggestionController: IQTextFieldSuggestionController? {
didSet {
if let controller = self.suggestionController {
controller.onSelectSuggestion = { [weak self] in self?._select(controller: $0, suggestion: $1) }
}
self.suggestionView.collectionController = self.suggestionController
}
}
public var onShouldBeginEditing: ShouldClosure?
public var onBeginEditing: Closure?
public var onEditing: Closure?
public var onShouldEndEditing: ShouldClosure?
public var onEndEditing: Closure?
public var onPressedAction: ActionClosure?
public var onShouldClear: ShouldClosure?
public var onPressedClear: Closure?
public var onShouldReturn: ShouldClosure?
public var onPressedReturn: Closure?
public var onSelectSuggestion: SelectSuggestionClosure?
open override var intrinsicContentSize: CGSize {
get { return self._field.intrinsicContentSize }
}
private var _field: Field!
private var _fieldDelegate: FieldDelegate!
private var _observer: QObserver< IQTextFieldObserver > = QObserver< IQTextFieldObserver >()
private lazy var _accessoryView: AccessoryView = AccessoryView(frame: CGRect(x: 0, y: 0, width: UIScreen.main.bounds.width, height: 0))
public required init() {
super.init(frame: CGRect(x: 0, y: 0, width: 100, height: 100))
}
public override init(frame: CGRect) {
super.init(frame: frame)
}
public required init?(coder: NSCoder) {
super.init(coder: coder)
}
open override func setup() {
super.setup()
self.backgroundColor = UIColor.clear
self._fieldDelegate = FieldDelegate(field: self)
self._field = Field(frame: self.bounds)
self._field.autoresizingMask = [ .flexibleWidth, .flexibleHeight ]
self._field.inputAccessoryView = self._accessoryView
self._field.delegate = self._fieldDelegate
self.addSubview(self._field)
}
public func add(observer: IQTextFieldObserver, priority: UInt) {
self._observer.add(observer, priority: priority)
}
public func remove(observer: IQTextFieldObserver) {
self._observer.remove(observer)
}
open func beginEditing() {
self._field.becomeFirstResponder()
}
open override func sizeThatFits(_ size: CGSize) -> CGSize {
return self._field.sizeThatFits(size)
}
open override func sizeToFit() {
return self._field.sizeToFit()
}
public func apply(_ styleSheet: QTextFieldStyleSheet) {
self.apply(styleSheet as QDisplayStyleSheet)
self.requireValidator = styleSheet.requireValidator
self.validator = styleSheet.validator
self.form = styleSheet.form
self.formatter = styleSheet.formatter
self.textInsets = styleSheet.textInsets
self.textStyle = styleSheet.textStyle
self.editingInsets = styleSheet.editingInsets
self.placeholderInsets = styleSheet.placeholderInsets
self.placeholder = styleSheet.placeholder
self.clearButtonMode = styleSheet.clearButtonMode
self.typingStyle = styleSheet.typingStyle
self.autocapitalizationType = styleSheet.autocapitalizationType
self.autocorrectionType = styleSheet.autocorrectionType
self.spellCheckingType = styleSheet.spellCheckingType
self.keyboardType = styleSheet.keyboardType
self.keyboardAppearance = styleSheet.keyboardAppearance
self.returnKeyType = styleSheet.returnKeyType
self.enablesReturnKeyAutomatically = styleSheet.enablesReturnKeyAutomatically
self.isSecureTextEntry = styleSheet.isSecureTextEntry
if #available(iOS 10.0, *) {
self.textContentType = styleSheet.textContentType
}
self.isEnabled = styleSheet.isEnabled
if let style = styleSheet.toolbarStyle {
self.toolbar.apply(style)
}
self.toolbarActions = styleSheet.toolbarActions
if let style = styleSheet.suggestionStyle {
self.suggestionView.apply(style)
}
self.suggestionController = styleSheet.suggestionController
self.suggestion = styleSheet.suggestion
}
}
// MARK: Private
private extension QTextField {
func _toolbarItems() -> [UIBarButtonItem] {
var items: [UIBarButtonItem] = []
if self.toolbarActions.isEmpty == false {
if self.toolbarActions.contains(.cancel) == true {
items.append(UIBarButtonItem(barButtonSystemItem: .cancel, target: self, action: #selector(self._pressedCancel(_:))))
}
items.append(UIBarButtonItem(barButtonSystemItem: .flexibleSpace, target: self, action: nil))
if self.toolbarActions.contains(.done) == true {
items.append(UIBarButtonItem(barButtonSystemItem: .done, target: self, action: #selector(self._pressedDone(_:))))
}
}
return items
}
func _updateAccessoryView() {
var height: CGFloat = 0
if self.toolbarActions.isEmpty == false {
self._accessoryView.addSubview(self.toolbar)
height += self.toolbar.frame.height
}
if self.suggestion != nil {
self._accessoryView.addSubview(self.suggestionView)
height += self.suggestionView.frame.height
}
let origin = self._accessoryView.frame.origin
let size = self._accessoryView.frame.size
if size.height != height {
self._accessoryView.frame = CGRect(
origin: origin,
size: CGSize(
width: size.width,
height: height
)
)
self._field.reloadInputViews()
}
}
func _select(controller: IQTextFieldSuggestionController, suggestion: String) {
self.suggestionController?.set(variants: [])
self._field.text = suggestion
self._field.sendActions(for: .editingChanged)
NotificationCenter.default.post(name: UITextField.textDidChangeNotification, object: self._field)
if let closure = self.onEditing {
closure(self)
}
self._observer.notify({ (observer) in
observer.editing(textField: self)
})
if let form = self.form {
form.validation()
}
if let closure = self.onSelectSuggestion {
closure(self, suggestion)
}
self._observer.notify({ (observer) in
observer.select(textField: self, suggestion: suggestion)
})
self.endEditing(false)
}
@objc
func _pressedCancel(_ sender: Any) {
self._pressed(action: .cancel)
self.endEditing(false)
}
@objc
func _pressedDone(_ sender: Any) {
self._pressed(action: .done)
self.endEditing(false)
}
func _pressed(action: QFieldAction) {
if let closure = self.onPressedAction {
closure(self, action)
}
self._observer.notify({ (observer) in
observer.pressed(textField: self, action: action)
})
}
}
// MARK: Private • AccessoryView
private extension QTextField {
class AccessoryView : UIView {
override func layoutSubviews() {
super.layoutSubviews()
let bounds = self.bounds
var offset: CGFloat = 0
for subview in self.subviews {
let height = subview.frame.height
subview.frame = CGRect(x: bounds.origin.x, y: offset, width: bounds.size.width, height: height)
offset += height
}
}
}
}
// MARK: Private • Field
private extension QTextField {
class Field : UITextField, IQView {
public var textInsets: UIEdgeInsets = UIEdgeInsets.zero
public var editingInsets: UIEdgeInsets?
public var placeholderInsets: UIEdgeInsets?
public override var inputAccessoryView: UIView? {
set(value) { super.inputAccessoryView = value }
get {
guard let view = super.inputAccessoryView else { return nil }
return view.isHidden == true ? nil : view
}
}
public override init(frame: CGRect) {
super.init(frame: frame)
self.setup()
}
public required init?(coder: NSCoder) {
super.init(coder: coder)
self.setup()
}
open func setup() {
self.backgroundColor = UIColor.clear
}
open override func textRect(forBounds bounds: CGRect) -> CGRect {
return bounds.inset(by: self.textInsets)
}
open override func editingRect(forBounds bounds: CGRect) -> CGRect {
guard let insets = self.editingInsets else {
return bounds.inset(by: self.textInsets)
}
return bounds.inset(by: insets)
}
open override func placeholderRect(forBounds bounds: CGRect) -> CGRect {
guard let insets = self.placeholderInsets else {
return bounds.inset(by: self.textInsets)
}
return bounds.inset(by: insets)
}
}
}
// MARK: Private • FieldDelegate
private extension QTextField {
class FieldDelegate : NSObject, UITextFieldDelegate {
public weak var field: QTextField?
private var _shouldReplacementString: Bool
public init(field: QTextField?) {
self.field = field
self._shouldReplacementString = false
super.init()
}
public func textFieldShouldBeginEditing(_ textField: UITextField) -> Bool {
guard let field = self.field, let closure = field.onShouldBeginEditing else { return true }
return closure(field)
}
public func textFieldDidBeginEditing(_ textField: UITextField) {
guard let field = self.field else { return }
if let suggestion = field.suggestion {
field.suggestionController?.set(variants: suggestion.variants(field.unformatText))
}
if let closure = field.onBeginEditing {
closure(field)
}
field._observer.notify({ (observer) in
observer.beginEditing(textField: field)
})
}
public func textFieldShouldEndEditing(_ textField: UITextField) -> Bool {
guard let field = self.field, let closure = field.onShouldEndEditing else { return true }
return closure(field)
}
public func textFieldDidEndEditing(_ textField: UITextField) {
guard let field = self.field else { return }
if let closure = field.onEndEditing {
closure(field)
}
field._observer.notify({ (observer) in
observer.endEditing(textField: field)
})
}
public func textField(_ textField: UITextField, shouldChangeCharactersIn range: NSRange, replacementString string: String) -> Bool {
guard let field = self.field else { return true }
if self._shouldReplacementString == false {
self._shouldReplacementString = true
var caretPosition: Int = range.location + string.count
var caretLength: Int = 0
var sourceText = textField.text ?? ""
if let sourceTextRange = sourceText.range(from: range) {
sourceText = sourceText.replacingCharacters(in: sourceTextRange, with: string)
}
var sourceUnformat: String
if let formatter = field.formatter {
sourceUnformat = formatter.unformat(sourceText, caret: &caretPosition)
} else {
sourceUnformat = sourceText
}
var autoCompleteVariants: [String] = []
if let suggestion = field.suggestion {
if sourceUnformat.count > 0 {
autoCompleteVariants = suggestion.variants(sourceUnformat)
}
if sourceUnformat.isEmpty == false && sourceUnformat.count <= caretPosition && string.count > 0 {
if let autoComplete = suggestion.autoComplete(sourceUnformat) {
caretLength = autoComplete.count - sourceUnformat.count
sourceUnformat = autoComplete
}
}
}
var isValid: Bool
if let validator = field.validator {
isValid = validator.validate(sourceUnformat).isValid
} else {
isValid = true
}
if field.requireValidator == false || string.isEmpty == true || isValid == true {
var selectionFrom: UITextPosition?
var selectionTo: UITextPosition?
if let formatter = field.formatter {
let format = formatter.format(sourceUnformat, caret: &caretPosition)
textField.text = format
selectionFrom = textField.position(from: textField.beginningOfDocument, offset: caretPosition)
selectionTo = textField.position(from: textField.beginningOfDocument, offset: caretPosition + caretLength)
} else {
textField.text = sourceUnformat
selectionFrom = textField.position(from: textField.beginningOfDocument, offset: caretPosition)
selectionTo = textField.position(from: textField.beginningOfDocument, offset: caretPosition + caretLength)
}
if let selectionFrom = selectionFrom, let selectionTo = selectionTo {
textField.selectedTextRange = textField.textRange(from: selectionFrom, to: selectionTo)
}
field.suggestionController?.set(variants: autoCompleteVariants)
textField.sendActions(for: .editingChanged)
NotificationCenter.default.post(name: UITextField.textDidChangeNotification, object: textField)
if let closure = field.onEditing {
closure(field)
}
field._observer.notify({ (observer) in
observer.editing(textField: field)
})
if let form = field.form {
form.validation()
}
}
self._shouldReplacementString = false
}
return false
}
public func textFieldShouldClear(_ textField: UITextField) -> Bool {
guard let field = self.field else { return true }
field.text = ""
if let shouldClosure = field.onShouldClear {
if shouldClosure(field) == true {
if let pressedClosure = field.onPressedClear {
pressedClosure(field)
}
field._observer.notify({ (observer) in
observer.pressedClear(textField: field)
})
}
} else {
if let pressedClosure = field.onPressedClear {
pressedClosure(field)
}
field._observer.notify({ (observer) in
observer.pressedClear(textField: field)
})
}
return true
}
public func textFieldShouldReturn(_ textField: UITextField) -> Bool {
guard let field = self.field else { return true }
if let shouldClosure = field.onShouldReturn {
if shouldClosure(field) == true {
if let pressedClosure = field.onPressedReturn {
pressedClosure(field)
}
field._observer.notify({ (observer) in
observer.pressedReturn(textField: field)
})
}
} else {
if let pressedClosure = field.onPressedReturn {
pressedClosure(field)
}
field._observer.notify({ (observer) in
observer.pressedReturn(textField: field)
})
}
return true
}
}
}
// MARK: UITextInputTraits
extension QTextField : UITextInputTraits {
public var autocapitalizationType: UITextAutocapitalizationType {
set(value) { self._field.autocapitalizationType = value }
get { return self._field.autocapitalizationType }
}
public var autocorrectionType: UITextAutocorrectionType {
set(value) { self._field.autocorrectionType = value }
get { return self._field.autocorrectionType }
}
public var spellCheckingType: UITextSpellCheckingType {
set(value) { self._field.spellCheckingType = value }
get { return self._field.spellCheckingType }
}
public var keyboardType: UIKeyboardType {
set(value) { self._field.keyboardType = value }
get { return self._field.keyboardType }
}
public var keyboardAppearance: UIKeyboardAppearance {
set(value) { self._field.keyboardAppearance = value }
get { return self._field.keyboardAppearance }
}
public var returnKeyType: UIReturnKeyType {
set(value) { self._field.returnKeyType = value }
get { return self._field.returnKeyType }
}
public var enablesReturnKeyAutomatically: Bool {
set(value) { self._field.enablesReturnKeyAutomatically = value }
get { return self._field.enablesReturnKeyAutomatically }
}
public var isSecureTextEntry: Bool {
set(value) { self._field.isSecureTextEntry = value }
get { return self._field.isSecureTextEntry }
}
@available(iOS 10.0, *)
public var textContentType: UITextContentType! {
set(value) { self._field.textContentType = value }
get { return self._field.textContentType }
}
}
| mit | 8cdd67d305f1454b6cdc68e81a755aef | 37.536806 | 140 | 0.594569 | 5.670388 | false | false | false | false |
audiokit/AudioKit | Sources/AudioKit/Internals/Hardware/AVAudioEngine+Devices.swift | 3 | 1550 | // Copyright AudioKit. All Rights Reserved. Revision History at http://github.com/AudioKit/AudioKit/
import AVFoundation
import Foundation
#if os(macOS)
extension AVAudioEngine {
func setDevice(id: AudioDeviceID) {
var outputID = id
if let outputUnit = outputNode.audioUnit {
let error = AudioUnitSetProperty(outputUnit,
kAudioOutputUnitProperty_CurrentDevice,
kAudioUnitScope_Global,
0,
&outputID,
UInt32(MemoryLayout<AudioDeviceID>.size))
if error != noErr {
Log("setDevice error: ", error)
}
}
}
func getDevice() -> AudioDeviceID {
if let outputUnit = outputNode.audioUnit {
var outputID: AudioDeviceID = 0
var propsize = UInt32(MemoryLayout<AudioDeviceID>.size)
let error = AudioUnitGetProperty(outputUnit,
kAudioOutputUnitProperty_CurrentDevice,
kAudioUnitScope_Global,
0,
&outputID,
&propsize)
if error != noErr {
Log("getDevice error: ", error)
}
return outputID
}
return 0
}
}
#endif
| mit | f0420d839351c475de138c8563b8e1ac | 35.904762 | 100 | 0.450323 | 6.512605 | false | false | false | false |
amoriello/trust-line-ios | Pods/CryptoSwift/CryptoSwift/NSDataExtension.swift | 4 | 2725 | //
// PGPDataExtension.swift
// SwiftPGP
//
// Created by Marcin Krzyzanowski on 05/07/14.
// Copyright (c) 2014 Marcin Krzyzanowski. All rights reserved.
//
import Foundation
extension NSMutableData {
/** Convenient way to append bytes */
internal func appendBytes(arrayOfBytes: [UInt8]) {
self.appendBytes(arrayOfBytes, length: arrayOfBytes.count)
}
}
extension NSData {
public func checksum() -> UInt16 {
var s:UInt32 = 0;
var bytesArray = self.arrayOfBytes()
for (var i = 0; i < bytesArray.count; i++) {
_ = bytesArray[i]
s = s + UInt32(bytesArray[i])
}
s = s % 65536;
return UInt16(s);
}
@nonobjc public func md5() -> NSData? {
return Hash.md5(self).calculate()
}
public func sha1() -> NSData? {
return Hash.sha1(self).calculate()
}
public func sha224() -> NSData? {
return Hash.sha224(self).calculate()
}
public func sha256() -> NSData? {
return Hash.sha256(self).calculate()
}
public func sha384() -> NSData? {
return Hash.sha384(self).calculate()
}
public func sha512() -> NSData? {
return Hash.sha512(self).calculate()
}
public func crc32() -> NSData? {
return Hash.crc32(self).calculate()
}
public func encrypt(cipher: Cipher) throws -> NSData? {
let encrypted = try cipher.encrypt(self.arrayOfBytes())
return NSData.withBytes(encrypted)
}
public func decrypt(cipher: Cipher) throws -> NSData? {
let decrypted = try cipher.decrypt(self.arrayOfBytes())
return NSData.withBytes(decrypted)
}
public func authenticate(authenticator: Authenticator) -> NSData? {
if let result = authenticator.authenticate(self.arrayOfBytes()) {
return NSData.withBytes(result)
}
return nil
}
}
extension NSData {
public func toHexString() -> String {
let count = self.length / sizeof(UInt8)
var bytesArray = [UInt8](count: count, repeatedValue: 0)
self.getBytes(&bytesArray, length:count * sizeof(UInt8))
var s:String = "";
for byte in bytesArray {
s = s + String(format:"%02x", byte)
}
return s
}
public func arrayOfBytes() -> [UInt8] {
let count = self.length / sizeof(UInt8)
var bytesArray = [UInt8](count: count, repeatedValue: 0)
self.getBytes(&bytesArray, length:count * sizeof(UInt8))
return bytesArray
}
class public func withBytes(bytes: [UInt8]) -> NSData {
return NSData(bytes: bytes, length: bytes.count)
}
}
| mit | ea1b0cf27d37b76b863b0dc9454cf190 | 24.707547 | 73 | 0.585321 | 4.205247 | false | false | false | false |
Bartlebys/Bartleby | Bartleby.xOS/core/extensions/BartlebyDocument+Collections.swift | 1 | 1954 | //
// BartlebyDocument+Collections.swift
// bartleby
//
// Created by Benoit Pereira da silva on 25/11/2016.
// Copyright © 2016 Benoit Pereira da silva. All rights reserved.
//
import Foundation
extension BartlebyDocument{
// MARK: - Collections Public API
open func getCollection<T: CollectibleCollection> () throws -> T {
guard let collection=self.collectionByName(T.collectionName) as? T else {
throw DocumentError.unExistingCollection(collectionName: T.collectionName)
}
return collection
}
/**
Returns the collection Names.
- returns: the names
*/
open func getCollectionsNames()->[String]{
return self._collections.map {$0.0}
}
// Any call should always be casted to a IterableCollectibleCollection
open func collectionByName(_ name: String) -> BartlebyCollection? {
if self._collections.keys.contains(name){
return self._collections[name]
}
return nil
}
// Weak Casting for internal behavior
// Those dynamic method are only used internally
internal func _addCollection(_ collection: BartlebyCollection) {
let collectionName=collection.d_collectionName
self._collections[collectionName]=collection
}
/**
Returns the collection file name
- parameter metadatum: the collectionMetadatim
- returns: the crypted and the non crypted file name in a tupple.
*/
internal func _collectionFileNames(_ metadatum: CollectionMetadatum) -> (notCrypted: String, crypted: String) {
let cryptedExtension=BartlebyDocument.DATA_EXTENSION
let nonCryptedExtension=".\(self.serializer.fileExtension)"
let cryptedFileName=metadatum.collectionName + cryptedExtension
let nonCryptedFileName=metadatum.collectionName + nonCryptedExtension
return (notCrypted:nonCryptedFileName, crypted:cryptedFileName)
}
}
| apache-2.0 | 897d0d12a939fe830e79d79125adff54 | 26.9 | 115 | 0.689196 | 4.919395 | false | false | false | false |
GreenvilleCocoa/ChristmasDelivery | ChristmasDelivery/GameOverScene.swift | 1 | 1448 | //
// GameOverScene.swift
// ChristmasDelivery
//
// Created by Marcus Smith on 12/8/14.
// Copyright (c) 2014 GreenvilleCocoaheads. All rights reserved.
//
import SpriteKit
class GameOverScene: SKScene {
let success: Bool
let level: Int
init(size: CGSize, success: Bool, level: Int) {
self.success = success
self.level = level
super.init(size: size)
}
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented, stop calling it")
}
override func didMoveToView(view: SKView) {
/* Setup your scene here */
let myLabel = SKLabelNode(fontNamed:"Chalkduster")
myLabel
if success {
myLabel.text = "Completed Level \(level)!";
}
else {
myLabel.text = "Game Over, score \(totalScore)!";
}
myLabel.fontSize = 44;
myLabel.position = CGPoint(x:CGRectGetMidX(self.frame), y:CGRectGetMidY(self.frame));
self.addChild(myLabel)
}
override func touchesBegan(touches: Set<UITouch>, withEvent event: UIEvent?) {
if !success {
totalScore = 0
}
let nextLevel: Int = success ? level + 1 : 1
let scene: GameScene = GameScene(size: size, level: nextLevel)
scene.scaleMode = .AspectFill
view!.presentScene(scene)
}
}
| mit | 6ebbec0f92f15f044ceb7c7174b5c208 | 24.857143 | 93 | 0.573895 | 4.553459 | false | false | false | false |
jsslai/Action | Carthage/Checkouts/RxSwift/Rx.playground/Pages/Combining_Operators.xcplaygroundpage/Contents.swift | 3 | 6027 | /*:
> # IMPORTANT: To use **Rx.playground**:
1. Open **Rx.xcworkspace**.
1. Build the **RxSwift-OSX** scheme (**Product** → **Build**).
1. Open **Rx** playground in the **Project navigator**.
1. Show the Debug Area (**View** → **Debug Area** → **Show Debug Area**).
----
[Previous](@previous) - [Table of Contents](Table_of_Contents)
*/
import RxSwift
/*:
# Combination Operators
Operators that combine multiple source `Observable`s into a single `Observable`.
## `startWith`
Emits the specified sequence of elements before beginning to emit the elements from the source `Observable`. [More info](http://reactivex.io/documentation/operators/startwith.html)
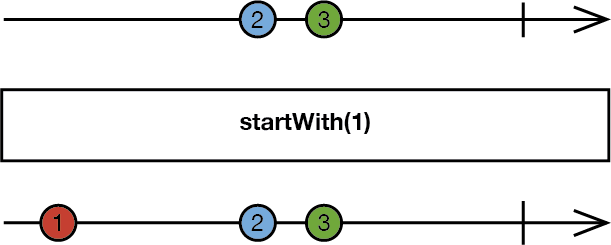
*/
example("startWith") {
let disposeBag = DisposeBag()
Observable.of("🐶", "🐱", "🐭", "🐹")
.startWith("1️⃣")
.startWith("2️⃣")
.startWith("3️⃣", "🅰️", "🅱️")
.subscribe(onNext: { print($0) })
.addDisposableTo(disposeBag)
}
/*:
> As this example demonstrates, `startWith` can be chained on a last-in-first-out basis, i.e., each successive `startWith`'s elements will be prepended before the prior `startWith`'s elements.
----
## `merge`
Combines elements from source `Observable` sequences into a single new `Observable` sequence, and will emit each element as it is emitted by each source `Observable` sequence. [More info](http://reactivex.io/documentation/operators/merge.html)
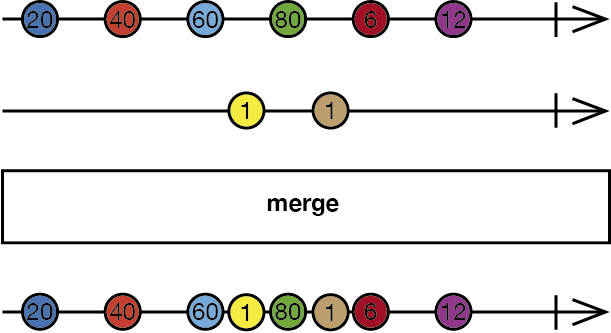
*/
example("merge") {
let disposeBag = DisposeBag()
let subject1 = PublishSubject<String>()
let subject2 = PublishSubject<String>()
Observable.of(subject1, subject2)
.merge()
.subscribe(onNext: { print($0) })
.addDisposableTo(disposeBag)
subject1.onNext("🅰️")
subject1.onNext("🅱️")
subject2.onNext("①")
subject2.onNext("②")
subject1.onNext("🆎")
subject2.onNext("③")
}
/*:
----
## `zip`
Combines up to 8 source `Observable` sequences into a single new `Observable` sequence, and will emit from the combined `Observable` sequence the elements from each of the source `Observable` sequences at the corresponding index. [More info](http://reactivex.io/documentation/operators/zip.html)
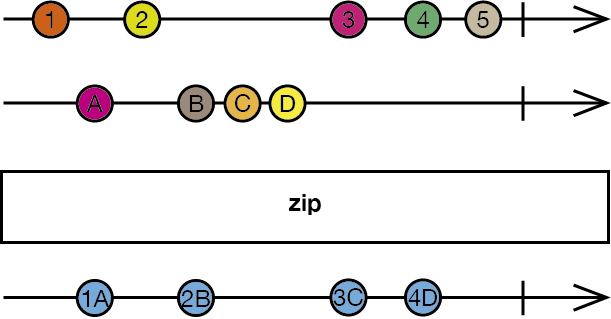
*/
example("zip") {
let disposeBag = DisposeBag()
let stringSubject = PublishSubject<String>()
let intSubject = PublishSubject<Int>()
Observable.zip(stringSubject, intSubject) { stringElement, intElement in
"\(stringElement) \(intElement)"
}
.subscribe(onNext: { print($0) })
.addDisposableTo(disposeBag)
stringSubject.onNext("🅰️")
stringSubject.onNext("🅱️")
intSubject.onNext(1)
intSubject.onNext(2)
stringSubject.onNext("🆎")
intSubject.onNext(3)
}
/*:
----
## `combineLatest`
Combines up to 8 source `Observable` sequences into a single new `Observable` sequence, and will begin emitting from the combined `Observable` sequence the latest elements of each source `Observable` sequence once all source sequences have emitted at least one element, and also when any of the source `Observable` sequences emits a new element. [More info](http://reactivex.io/documentation/operators/combinelatest.html)

*/
example("combineLatest") {
let disposeBag = DisposeBag()
let stringSubject = PublishSubject<String>()
let intSubject = PublishSubject<Int>()
Observable.combineLatest(stringSubject, intSubject) { stringElement, intElement in
"\(stringElement) \(intElement)"
}
.subscribe(onNext: { print($0) })
.addDisposableTo(disposeBag)
stringSubject.onNext("🅰️")
stringSubject.onNext("🅱️")
intSubject.onNext(1)
intSubject.onNext(2)
stringSubject.onNext("🆎")
}
//: There is also a `combineLatest` extension on `Array`:
example("Array.combineLatest") {
let disposeBag = DisposeBag()
let stringObservable = Observable.just("❤️")
let fruitObservable = Observable.from(["🍎", "🍐", "🍊"])
let animalObservable = Observable.of("🐶", "🐱", "🐭", "🐹")
[stringObservable, fruitObservable, animalObservable].combineLatest {
"\($0[0]) \($0[1]) \($0[2])"
}
.subscribe(onNext: { print($0) })
.addDisposableTo(disposeBag)
}
/*:
> The `combineLatest` extension on `Array` requires that all source `Observable` sequences are of the same type.
----
## `switchLatest`
Transforms the elements emitted by an `Observable` sequence into `Observable` sequences, and emits elements from the most recent inner `Observable` sequence. [More info](http://reactivex.io/documentation/operators/switch.html)

*/
example("switchLatest") {
let disposeBag = DisposeBag()
let subject1 = BehaviorSubject(value: "⚽️")
let subject2 = BehaviorSubject(value: "🍎")
let variable = Variable(subject1)
variable.asObservable()
.switchLatest()
.subscribe(onNext: { print($0) })
.addDisposableTo(disposeBag)
subject1.onNext("🏈")
subject1.onNext("🏀")
variable.value = subject2
subject1.onNext("⚾️")
subject2.onNext("🍐")
}
/*:
> In this example, adding ⚾️ onto `subject1` after setting `variable.value` to `subject2` has no effect, because only the most recent inner `Observable` sequence (`subject2`) will emit elements.
*/
//: [Next](@next) - [Table of Contents](Table_of_Contents)
| mit | 813e7690f64a3ae5812fc928e50028b2 | 35.83125 | 422 | 0.667911 | 4.371662 | false | true | false | false |
benwwchen/sysujwxt-ios | SYSUJwxt/NotifySettingTableViewController.swift | 1 | 7685 | //
// NotifySettingTableViewController.swift
// SYSUJwxt
//
// Created by benwwchen on 2017/8/24.
// Copyright © 2017年 benwwchen. All rights reserved.
//
import UIKit
import os.log
class NotifySettingTableViewController: UITableViewController, UIPickerViewDelegate, UIPickerViewDataSource {
// MARK: Properties
var year: String = ""
var term: String = ""
var isNotifyOn: Bool = UserDefaults.standard.bool(forKey: "notify.isOn")
// choices
var years = [String]()
// editing
var lastSelectedYear: String = ""
var pickerView: UIPickerView?
@IBOutlet weak var yearTableViewCell: UITableViewCell!
@IBOutlet weak var yearLabel: UILabel!
@IBOutlet weak var yearTextField: UITextField!
@IBOutlet weak var saveButton: UIBarButtonItem!
@IBOutlet weak var notifySwitch: UISwitch!
@IBOutlet weak var termSegmentedControl: UISegmentedControl!
override func viewDidLoad() {
super.viewDidLoad()
// load saved settings
// try to get the saved year
if let savedYear = UserDefaults.standard.object(forKey: "notify.year") as? String {
year = savedYear
} else {
// no saved value, init it as the current year and save it
let dateFormatter = DateFormatter()
dateFormatter.dateFormat = "yyyy"
if let yearInt = Int(dateFormatter.string(from: Date())) {
year = "\(yearInt)-\(yearInt+1)"
}
}
// try to get the saved term
if let savedTerm = UserDefaults.standard.object(forKey: "notify.term") as? String {
term = savedTerm
} else {
// no saved value, init it as the current term and save it
let dateFormatter = DateFormatter()
dateFormatter.dateFormat = "mm"
if let month = Int(dateFormatter.string(from: Date())),
month >= 8 || month <= 2 {
term = "1"
} else {
term = "2"
}
}
// switch
notifySwitch.isOn = isNotifyOn
// setup the picker for year selector
initPickerData()
initPickerView()
// populate the data
yearLabel.text = year
termSegmentedControl.selectedSegmentIndex = Int(term)! - 1
}
// year picker
func initPickerData() {
let dateFormatter = DateFormatter()
dateFormatter.dateFormat = "yyyy"
if let currentYear = Int(dateFormatter.string(from: Date())) {
let entranceYear = JwxtApiClient.shared.grade
for year in (entranceYear...currentYear).reversed() {
years.append("\(year)-\(year+1)")
}
}
}
func initPickerView() {
let newPickerView = UIPickerView(frame: CGRect(x: 0, y: 0, width: self.view.frame.size.width, height: self.view.frame.size.height / 3.0))
newPickerView.backgroundColor = .white
newPickerView.showsSelectionIndicator = true
newPickerView.delegate = self
newPickerView.dataSource = self
newPickerView.selectRow(years.index(of: year)!, inComponent: 0, animated: false)
pickerView = newPickerView
let toolBar = UIToolbar()
toolBar.barStyle = .default
toolBar.isTranslucent = true
toolBar.sizeToFit()
let doneButton = UIBarButtonItem(barButtonSystemItem: UIBarButtonSystemItem.done, target: self, action: #selector(donePicker))
let spaceButton = UIBarButtonItem(barButtonSystemItem: UIBarButtonSystemItem.flexibleSpace, target: nil, action: nil)
let cancelButton = UIBarButtonItem(barButtonSystemItem: UIBarButtonSystemItem.cancel, target: self, action: #selector(cancelPicker))
toolBar.setItems([cancelButton, spaceButton, doneButton], animated: false)
toolBar.isUserInteractionEnabled = true
yearTextField.inputView = pickerView
yearTextField.inputAccessoryView = toolBar
}
// MARK: - Picker view data source
func pickerView(_ pickerView: UIPickerView, numberOfRowsInComponent component: Int) -> Int {
return years.count
}
func pickerView(_ pickerView: UIPickerView, didSelectRow row: Int, inComponent component: Int) {
year = years[row]
yearLabel.text = year
yearTextField.text = year
}
func pickerView(_ pickerView: UIPickerView, titleForRow row: Int, forComponent component: Int) -> String? {
return years[row]
}
func numberOfComponents(in pickerView: UIPickerView) -> Int {
return 1
}
func donePicker(sender: UIBarButtonItem) {
yearTextField.resignFirstResponder()
yearTableViewCell.setSelected(false, animated: true)
}
func cancelPicker(sender: UIBarButtonItem) {
yearTextField.resignFirstResponder()
yearTableViewCell.setSelected(false, animated: true)
year = lastSelectedYear
yearTextField.text = year
yearLabel.text = year
}
@IBAction func termSegmentedControlValueChanged(_ sender: UISegmentedControl) {
if let title = sender.titleForSegment(at: sender.selectedSegmentIndex) {
term = title
}
}
// MARK: - Table view data source
override func numberOfSections(in tableView: UITableView) -> Int {
if isNotifyOn {
return 2
}
return 1
}
override func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
// show picker for year selection
if let cell = tableView.cellForRow(at: indexPath), cell.reuseIdentifier == "yearCell" {
lastSelectedYear = year
yearTextField.becomeFirstResponder()
}
}
// MARK: Actions
@IBAction func cancelButtonPressed(_ sender: UIBarButtonItem) {
dismiss(animated: true, completion: nil)
}
@IBAction func switchValueChanged(_ sender: UISwitch) {
isNotifyOn = sender.isOn
if sender.isOn {
LocalNotification.registerForLocalNotification(on: UIApplication.shared, currentViewController: self)
}
tableView.reloadData()
}
override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
super.prepare(for: segue, sender: sender)
// Configure the destination view controller only when the save button is pressed.
if let button = sender as? UIBarButtonItem, button === saveButton {
UserDefaults.standard.set(isNotifyOn, forKey: "notify.isOn")
UserDefaults.standard.set(year, forKey: "notify.year")
UserDefaults.standard.set(term, forKey: "notify.term")
if isNotifyOn {
// check updates every 2 hours
UIApplication.shared.setMinimumBackgroundFetchInterval(2400)
} else {
UIApplication.shared.setMinimumBackgroundFetchInterval(UIApplicationBackgroundFetchIntervalNever)
}
} else {
if #available(iOS 10.0, *) {
os_log("The save button was not pressed, cancelling", log: OSLog.default, type: .debug)
} else {
// Fallback on earlier versions
}
}
}
}
extension UITableView {
func reloadData(with animation: UITableViewRowAnimation) {
reloadSections(IndexSet(integersIn: 0..<numberOfSections), with: animation)
}
}
| bsd-3-clause | 23bd2aac4ded6fd2bc334a21dc9191ca | 33.142222 | 145 | 0.614033 | 5.364525 | false | false | false | false |
cdtschange/SwiftMKit | SwiftMKit/UI/View/Button/IndicatorButton.swift | 1 | 5891 | //
// IndicatorButton.swift
// SwiftMKitDemo
//
// Created by apple on 5/17/16.
// Copyright © 2016 cdts. All rights reserved.
//
import UIKit
open class IndicatorButton: UIButton {
public enum AnimateDirection: Int {
case fromDownToUp, fromUpToDown
}
public enum IndicatorPosition: Int {
case left, right
}
// MARK: - 公开属性
/// 标识是否是向下切换title
public var animateDirection: AnimateDirection = .fromDownToUp
public var indicatorStyle: UIActivityIndicatorViewStyle = .white {
didSet {
indicatorView.activityIndicatorViewStyle = indicatorStyle
}
}
public var indicatorPosition: IndicatorPosition = .left
public var indicatorMargin: CGFloat = 8
open override var isEnabled: Bool {
willSet {
if newValue != isEnabled {
Async.main {
if let title = self.title(for: .disabled) {
if title.length > 0 {
self.disabledTitle = title
self.setTitle("", for: .disabled)
}
}
}
}
}
didSet {
if oldValue != isEnabled {
Async.main {
if oldValue {
// 动画切换title,显示菊花
self.ib_loadingWithTitle(self.disabledTitle)
} else {
// 重置按钮,隐藏菊花
self.ib_resetToNormalState()
}
}
}
}
}
// MARK: - 私有属性
lazy var backView = UIView()
lazy var lblMessage = UILabel()
lazy var indicatorView = UIActivityIndicatorView()
fileprivate var lastTitle: String?
fileprivate var disabledTitle: String?
fileprivate var fastEnabled: Bool = false
fileprivate var transformY: CGFloat {
get {
return self.h * (animateDirection == .fromDownToUp ? 1 : -1)
}
}
// MARK: - 构造方法
required public init?(coder decoder: NSCoder) {
super.init(coder: decoder)
setup()
}
init() {
super.init(frame: CGRect.zero)
setup()
}
// MARK: - 私有方法
fileprivate func setup() {
layer.masksToBounds = true
// 初始化backView及其子视图
lblMessage.textColor = titleLabel?.textColor
lblMessage.font = titleLabel?.font
lblMessage.textAlignment = .center
backView.addSubview(lblMessage)
indicatorView.activityIndicatorViewStyle = indicatorStyle
indicatorView.hidesWhenStopped = true
indicatorView.sizeToFit()
backView.addSubview(indicatorView)
// 要先设置高度 再设置center
backView.h = self.h
backView.center = CGPoint(x: self.w * 0.5, y: self.h * 0.5)
backView.backgroundColor = UIColor.clear
backView.alpha = 0
addSubview(backView)
lastTitle = currentTitle
disabledTitle = title(for: .disabled)
self.setTitle("", for: .disabled)
}
fileprivate func ib_loadingWithTitle(_ title: String?) {
let color = titleColor(for: .disabled)
let shadowColor = titleShadowColor(for: .disabled)
lblMessage.text = title
lblMessage.textColor = color
lblMessage.shadowColor = shadowColor
lblMessage.sizeToFit()
// 计算lblMessage 和 indicatorView 的位置
indicatorView.centerY = backView.centerY
lblMessage.centerY = indicatorView.centerY
switch indicatorPosition {
case .left:
lblMessage.left = indicatorView.right + indicatorMargin
backView.right = lblMessage.right
case .right:
indicatorView.left = lblMessage.right + indicatorMargin
backView.right = indicatorView.right
}
backView.w = indicatorView.w + indicatorMargin + lblMessage.w
backView.left = (self.w - backView.w) * 0.5
if backView.left < 0 { // 文字太长
backView.w = lblMessage.w
lblMessage.left = 0
if backView.w > self.w {
lblMessage.w = self.w
lblMessage.adjustsFontSizeToFitWidth = true
backView.w = lblMessage.w
}
backView.left = (self.w - backView.w) * 0.5
} else {
indicatorView.startAnimating()
}
if title == lastTitle {
// 如果title和旧title相同 不需要显示动画滚动
} else {
backView.transform = CGAffineTransform(translationX: 0, y: transformY)
}
fastEnabled = false
Async.main(after: 0.1) {
if self.fastEnabled {
return
}
UIView.animate(withDuration: 0.2, animations: {
self.titleLabel!.alpha = 0
self.backView.alpha = 1
self.backView.transform = CGAffineTransform.identity
})
}
}
fileprivate func ib_resetToNormalState() {
fastEnabled = true
UIView.animate(withDuration: 0.2, animations: {
self.titleLabel!.alpha = 1
self.backView.alpha = 0
if self.currentTitle == self.disabledTitle {
// 如果title和旧title相同 不需要显示动画滚动
} else {
self.backView.transform = CGAffineTransform(translationX: 0, y: self.transformY)
}
}, completion: { (finished) in
if self.isEnabled {
self.backView.transform = CGAffineTransform.identity
self.indicatorView.stopAnimating()
}
})
}
}
| mit | 3c4f130e6df4d7c4e7e3a2c74ba75b83 | 30.966292 | 96 | 0.547803 | 5.089445 | false | false | false | false |
ypopovych/Boilerplate | Sources/Boilerplate/AnyContainer.swift | 2 | 1428 | //===--- AnyContainer.swift ------------------------------------------------------===//
//Copyright (c) 2016 Daniel Leping (dileping)
//
//Licensed under the Apache License, Version 2.0 (the "License");
//you may not use this file except in compliance with the License.
//You may obtain a copy of the License at
//
//http://www.apache.org/licenses/LICENSE-2.0
//
//Unless required by applicable law or agreed to in writing, software
//distributed under the License is distributed on an "AS IS" BASIS,
//WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
//See the License for the specific language governing permissions and
//limitations under the License.
//===----------------------------------------------------------------------===//
import Foundation
//useful for C-interoperability when you need to pass non-AnyObject inside C
open class AnyContainer<T> : ContainerType {
public typealias Value = T
internal (set) public var content:T
public init(_ content:T) {
self.content = content
}
public func withdraw() throws -> Value {
return content
}
}
open class MutableAnyContainer<T> : AnyContainer<T> {
public override var content:T {
get {
return super.content
}
set {
super.content = newValue
}
}
public override init(_ content:T) {
super.init(content)
}
}
| apache-2.0 | 8d8ebeb64975b0ac07d71796ef5ee36e | 29.382979 | 87 | 0.602241 | 4.697368 | false | false | false | false |
tuanan94/FRadio-ios | Pods/NotificationBannerSwift/NotificationBanner/Classes/StatusBarNotificationBanner.swift | 1 | 3099 | /*
The MIT License (MIT)
Copyright (c) 2017 Dalton Hinterscher
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"),
to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense,
and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR
ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH
THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*/
import UIKit
#if CARTHAGE_CONFIG
import MarqueeLabelSwift
#else
import MarqueeLabel
#endif
public class StatusBarNotificationBanner: BaseNotificationBanner {
public override var bannerHeight: CGFloat {
get {
if let customBannerHeight = customBannerHeight {
return customBannerHeight
} else if shouldAdjustForIphoneX() {
return super.bannerHeight
} else {
return 20.0
}
} set {
customBannerHeight = newValue
}
}
override init(style: BannerStyle, colors: BannerColorsProtocol? = nil) {
super.init(style: style, colors: colors)
titleLabel = MarqueeLabel()
titleLabel?.animationDelay = 2
titleLabel?.type = .leftRight
titleLabel!.font = UIFont.systemFont(ofSize: 12.5, weight: UIFont.Weight.bold)
titleLabel!.textAlignment = .center
titleLabel!.textColor = .white
contentView.addSubview(titleLabel!)
titleLabel!.snp.makeConstraints { (make) in
make.top.equalToSuperview()
make.left.equalToSuperview().offset(5)
make.right.equalToSuperview().offset(-5)
make.bottom.equalToSuperview()
}
updateMarqueeLabelsDurations()
}
public convenience init(title: String,
style: BannerStyle = .info,
colors: BannerColorsProtocol? = nil) {
self.init(style: style, colors: colors)
titleLabel!.text = title
}
public convenience init(attributedTitle: NSAttributedString,
style: BannerStyle = .info,
colors: BannerColorsProtocol? = nil) {
self.init(style: style, colors: colors)
titleLabel!.attributedText = attributedTitle
}
required public init?(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)
}
}
| mit | 9bce21fec14dfb095515176a4bb268a6 | 36.792683 | 147 | 0.660213 | 5.234797 | false | false | false | false |
kreshikhin/scituner | SciTuner/Views/TuningView.swift | 1 | 3669 | //
// TuningView.swift
// SciTuner
//
// Created by Denis Kreshikhin on 8/16/17.
// Copyright © 2017 Denis Kreshikhin. All rights reserved.
//
import UIKit
import CoreText
class TuningView: UIView {
var labels: [UILabel] = []
let stackView = UIStackView()
var pointerView = PointerView(frame: CGRect())
let defaultMargins = UIEdgeInsets(top: 20, left: 20, bottom: 20, right: 20)
var tuning: Tuning? {
didSet {
labels.forEach { $0.removeFromSuperview() }
labels.removeAll()
for note in tuning?.strings ?? [] {
let label = UILabel()
label.backgroundColor = .red
label.text = note.string
labels.append(label)
label.translatesAutoresizingMaskIntoConstraints = false
label.heightAnchor.constraint(equalTo: label.widthAnchor).isActive = true
label.textColor = UIColor.white
label.backgroundColor = UIColor.clear
stackView.addArrangedSubview(label)
}
}
}
var notePosition: CGFloat = 0 {
didSet {
let height = frame.size.height / 2
let firstCenterX = labels.first?.center.x ?? 0
let lastCenterX = labels.last?.center.x ?? frame.size.width
if let count = tuning?.strings.count {
let step = (lastCenterX - firstCenterX) / CGFloat(count - 1)
var shift = notePosition
//print("noteposition", notePosition)
if notePosition < 0.0 {
shift = 0.5 * exp(notePosition) - 0.5
}
if notePosition > CGFloat(count) - 1.0 {
shift = CGFloat(count) - 0.5 * exp(-notePosition+CGFloat(count)-1)
}
pointerView.frame.size = CGSize(width: height, height: height)
pointerView.layer.cornerRadius = height / 2
pointerView.center.x = CGFloat(shift) * step + firstCenterX
pointerView.center.y = frame.size.height / 2
}
}
}
override init(frame: CGRect) {
super.init(frame: frame)
stackView.axis = .horizontal
stackView.distribution = .equalCentering
translatesAutoresizingMaskIntoConstraints = false
heightAnchor.constraint(equalTo: self.widthAnchor, multiplier: 0.2).isActive = true
addSubview(stackView)
stackView.translatesAutoresizingMaskIntoConstraints = false
stackView.widthAnchor.constraint(equalTo: self.widthAnchor).isActive = true
stackView.heightAnchor.constraint(equalTo: self.heightAnchor).isActive = true
stackView.topAnchor.constraint(equalTo: self.topAnchor).isActive = true
stackView.leftAnchor.constraint(equalTo: self.leftAnchor).isActive = true
stackView.layoutMargins = defaultMargins
stackView.isLayoutMarginsRelativeArrangement = true
addSubview(pointerView)
hidePointer()
}
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
func hidePointer(){
UIView.animate(withDuration: 0.100) {
self.pointerView.alpha = 0.0
}
}
func showPointer(){
UIView.animate(withDuration: 0.100) {
self.pointerView.alpha = 1.0
}
}
}
| mit | 4494ad89897f5c9b2ae593ecc0d55f3e | 32.045045 | 91 | 0.554526 | 5.277698 | false | false | false | false |
brentdax/swift | test/Compatibility/protocol_composition.swift | 5 | 2123 | // RUN: %empty-directory(%t)
// RUN: %{python} %utils/split_file.py -o %t %s
// RUN: %target-swift-frontend -typecheck -primary-file %t/swift4.swift %t/common.swift -verify -swift-version 4
// BEGIN common.swift
protocol P1 {
static func p1() -> Int
}
protocol P2 {
static var p2: Int { get }
}
// BEGIN swift3.swift
// Warning for mistakenly accepted protocol composition production.
func foo(x: P1 & Any & P2.Type?) {
// expected-warning @-1 {{protocol-constrained type with postfix '.Type' is ambiguous and will be rejected in future version of Swift}} {{13-13=(}} {{26-26=)}}
let _: (P1 & P2).Type? = x
let _: (P1 & P2).Type = x!
let _: Int = x!.p1()
let _: Int? = x?.p2
}
// Type expression
func bar() -> ((P1 & P2)?).Type {
let x = (P1 & P2?).self
// expected-warning @-1 {{protocol-constrained type with postfix '?' is ambiguous and will be rejected in future version of Swift}} {{12-12=(}} {{19-19=)}}
return x
}
// Non-ident type at non-last position are rejected anyway.
typealias A1 = P1.Type & P2 // expected-error {{non-protocol, non-class type 'P1.Type' cannot be used within a protocol-constrained type}}
// BEGIN swift4.swift
func foo(x: P1 & Any & P2.Type?) { // expected-error {{non-protocol, non-class type 'P2.Type?' cannot be used within a protocol-constrained type}}
let _: (P1 & P2).Type? = x // expected-error {{cannot convert value of type 'P1' to specified type '(P1 & P2).Type?'}}
let _: (P1 & P2).Type = x! // expected-error {{cannot force unwrap value of non-optional type 'P1'}}
let _: Int = x!.p1() // expected-error {{cannot force unwrap value of non-optional type 'P1'}}
let _: Int? = x?.p2 // expected-error {{cannot use optional chaining on non-optional value of type 'P1'}}
}
func bar() -> ((P1 & P2)?).Type {
let x = (P1 & P2?).self // expected-error {{non-protocol, non-class type 'P2?' cannot be used within a protocol-constrained type}}
return x // expected-error {{cannot convert return expression}}
}
typealias A1 = P1.Type & P2 // expected-error {{non-protocol, non-class type 'P1.Type' cannot be used within a protocol-constrained type}}
| apache-2.0 | eda510b403660e4aaa16afaed765d2d0 | 43.229167 | 161 | 0.661328 | 3.197289 | false | false | false | false |
Pandanx/PhotoPickerController | Classes/PhotoGridCell.swift | 1 | 2929 | //
// PhotoGridCell.swift
// Photos
//
// Created by 张晓鑫 on 2017/6/1.
// Copyright © 2017年 Wang. All rights reserved.
//
import UIKit
class PhotoGridCell: UICollectionViewCell {
// @IBOutlet weak var imageView: UIImageView!
// @IBOutlet weak var selectedImageView: UIImageView!
var imageView: UIImageView! = UIImageView()
var selectedImageView: UIImageView! = UIImageView()
var assistantLabel: UILabel = UILabel()
open override var isSelected: Bool {
didSet{
if isSelected {
selectedImageView.image = UIImage(named:"CellBlueSelected")
} else {
selectedImageView.image = UIImage(named:"CellGreySelected")
}
}
}
var duration: TimeInterval = 0 {
didSet {
let newValue = Int(ceil(duration))
assistantLabel.text = String(format: "%d:%02d", arguments: [newValue / 60, newValue % 60])
assistantLabel.isHidden = false
}
}
override init(frame: CGRect) {
super.init(frame: frame)
imageView.frame = self.bounds
imageView.contentMode = .scaleAspectFill
imageView.clipsToBounds = true
self.addSubview(imageView)
selectedImageView.frame = CGRect(x: self.bounds.width - 35, y: 0, width: 30, height: 30)
selectedImageView.image = UIImage.init(named: "CellGreySelected")
self.addSubview(selectedImageView)
assistantLabel.textColor = #colorLiteral(red: 1.0, green: 1.0, blue: 1.0, alpha: 1.0)
self.addSubview(assistantLabel)
assistantLabel.isHidden = true
assistantLabel.translatesAutoresizingMaskIntoConstraints = false
self.addConstraint(NSLayoutConstraint(item: self, attribute: .trailing, relatedBy: .equal, toItem: assistantLabel, attribute: .trailing, multiplier: 1, constant: 8))
self.addConstraint(NSLayoutConstraint(item: self, attribute: .bottom, relatedBy: .equal, toItem: assistantLabel, attribute: .bottom, multiplier: 1, constant: 8))
}
override func prepareForReuse() {
super.prepareForReuse()
assistantLabel.isHidden = true
}
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
func showAnim() {
UIView.animateKeyframes(withDuration: 0.4, delay: 0, options: UIViewKeyframeAnimationOptions.allowUserInteraction, animations: {
UIView.addKeyframe(withRelativeStartTime: 0, relativeDuration: 0.2, animations: {
self.selectedImageView.transform = CGAffineTransform(scaleX: 0.7, y:0.7)
})
UIView.addKeyframe(withRelativeStartTime: 0.2, relativeDuration: 0.4, animations: {
self.selectedImageView.transform = CGAffineTransform.identity
})
}, completion: nil)
}
}
| mit | 0f6c6a2070f14d935998915153fd4a86 | 36.922078 | 173 | 0.639384 | 4.891122 | false | false | false | false |
davejlin/treehouse | swift/swift2/fotofun/Pods/ReactiveCocoa/ReactiveCocoa/Swift/Action.swift | 22 | 6156 | import Foundation
import enum Result.NoError
/// Represents an action that will do some work when executed with a value of
/// type `Input`, then return zero or more values of type `Output` and/or fail
/// with an error of type `Error`. If no failure should be possible, NoError can
/// be specified for the `Error` parameter.
///
/// Actions enforce serial execution. Any attempt to execute an action multiple
/// times concurrently will return an error.
public final class Action<Input, Output, Error: ErrorType> {
private let executeClosure: Input -> SignalProducer<Output, Error>
private let eventsObserver: Signal<Event<Output, Error>, NoError>.Observer
/// A signal of all events generated from applications of the Action.
///
/// In other words, this will send every `Event` from every signal generated
/// by each SignalProducer returned from apply().
public let events: Signal<Event<Output, Error>, NoError>
/// A signal of all values generated from applications of the Action.
///
/// In other words, this will send every value from every signal generated
/// by each SignalProducer returned from apply().
public let values: Signal<Output, NoError>
/// A signal of all errors generated from applications of the Action.
///
/// In other words, this will send errors from every signal generated by
/// each SignalProducer returned from apply().
public let errors: Signal<Error, NoError>
/// Whether the action is currently executing.
public var executing: AnyProperty<Bool> {
return AnyProperty(_executing)
}
private let _executing: MutableProperty<Bool> = MutableProperty(false)
/// Whether the action is currently enabled.
public var enabled: AnyProperty<Bool> {
return AnyProperty(_enabled)
}
private let _enabled: MutableProperty<Bool> = MutableProperty(false)
/// Whether the instantiator of this action wants it to be enabled.
private let userEnabled: AnyProperty<Bool>
/// This queue is used for read-modify-write operations on the `_executing`
/// property.
private let executingQueue = dispatch_queue_create("org.reactivecocoa.ReactiveCocoa.Action.executingQueue", DISPATCH_QUEUE_SERIAL)
/// Whether the action should be enabled for the given combination of user
/// enabledness and executing status.
private static func shouldBeEnabled(userEnabled userEnabled: Bool, executing: Bool) -> Bool {
return userEnabled && !executing
}
/// Initializes an action that will be conditionally enabled, and create a
/// SignalProducer for each input.
public init<P: PropertyType where P.Value == Bool>(enabledIf: P, _ execute: Input -> SignalProducer<Output, Error>) {
executeClosure = execute
userEnabled = AnyProperty(enabledIf)
(events, eventsObserver) = Signal<Event<Output, Error>, NoError>.pipe()
values = events.map { $0.value }.ignoreNil()
errors = events.map { $0.error }.ignoreNil()
_enabled <~ enabledIf.producer
.combineLatestWith(_executing.producer)
.map(Action.shouldBeEnabled)
}
/// Initializes an action that will be enabled by default, and create a
/// SignalProducer for each input.
public convenience init(_ execute: Input -> SignalProducer<Output, Error>) {
self.init(enabledIf: ConstantProperty(true), execute)
}
deinit {
eventsObserver.sendCompleted()
}
/// Creates a SignalProducer that, when started, will execute the action
/// with the given input, then forward the results upon the produced Signal.
///
/// If the action is disabled when the returned SignalProducer is started,
/// the produced signal will send `ActionError.NotEnabled`, and nothing will
/// be sent upon `values` or `errors` for that particular signal.
@warn_unused_result(message="Did you forget to call `start` on the producer?")
public func apply(input: Input) -> SignalProducer<Output, ActionError<Error>> {
return SignalProducer { observer, disposable in
var startedExecuting = false
dispatch_sync(self.executingQueue) {
if self._enabled.value {
self._executing.value = true
startedExecuting = true
}
}
if !startedExecuting {
observer.sendFailed(.NotEnabled)
return
}
self.executeClosure(input).startWithSignal { signal, signalDisposable in
disposable.addDisposable(signalDisposable)
signal.observe { event in
observer.action(event.mapError(ActionError.ProducerError))
self.eventsObserver.sendNext(event)
}
}
disposable += {
self._executing.value = false
}
}
}
}
public protocol ActionType {
/// The type of argument to apply the action to.
associatedtype Input
/// The type of values returned by the action.
associatedtype Output
/// The type of error when the action fails. If errors aren't possible then `NoError` can be used.
associatedtype Error: ErrorType
/// Whether the action is currently enabled.
var enabled: AnyProperty<Bool> { get }
/// Extracts an action from the receiver.
var action: Action<Input, Output, Error> { get }
/// Creates a SignalProducer that, when started, will execute the action
/// with the given input, then forward the results upon the produced Signal.
///
/// If the action is disabled when the returned SignalProducer is started,
/// the produced signal will send `ActionError.NotEnabled`, and nothing will
/// be sent upon `values` or `errors` for that particular signal.
func apply(input: Input) -> SignalProducer<Output, ActionError<Error>>
}
extension Action: ActionType {
public var action: Action {
return self
}
}
/// The type of error that can occur from Action.apply, where `Error` is the type of
/// error that can be generated by the specific Action instance.
public enum ActionError<Error: ErrorType>: ErrorType {
/// The producer returned from apply() was started while the Action was
/// disabled.
case NotEnabled
/// The producer returned from apply() sent the given error.
case ProducerError(Error)
}
public func == <Error: Equatable>(lhs: ActionError<Error>, rhs: ActionError<Error>) -> Bool {
switch (lhs, rhs) {
case (.NotEnabled, .NotEnabled):
return true
case let (.ProducerError(left), .ProducerError(right)):
return left == right
default:
return false
}
}
| unlicense | 5214ef7b55de4ccf4a5111fd5886e0de | 33.977273 | 131 | 0.73538 | 4.151045 | false | false | false | false |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.