repo
stringclasses 856
values | pull_number
int64 3
127k
| instance_id
stringlengths 12
58
| issue_numbers
sequencelengths 1
5
| base_commit
stringlengths 40
40
| patch
stringlengths 67
1.54M
| test_patch
stringlengths 0
107M
| problem_statement
stringlengths 3
307k
| hints_text
stringlengths 0
908k
| created_at
timestamp[s] |
---|---|---|---|---|---|---|---|---|---|
streamlink/streamlink | 5,096 | streamlink__streamlink-5096 | [
"5080"
] | 871f7f7b3a438d7c901e4ba43ba8c64b6657dbf0 | diff --git a/src/streamlink/plugins/mixcloud.py b/src/streamlink/plugins/mixcloud.py
new file mode 100644
--- /dev/null
+++ b/src/streamlink/plugins/mixcloud.py
@@ -0,0 +1,76 @@
+"""
+$description British music live-streaming platform for radio shows and DJ mixes.
+$url mixcloud.com
+$type live
+"""
+
+import logging
+import re
+
+from streamlink.plugin import Plugin, pluginmatcher
+from streamlink.plugin.api import validate
+from streamlink.stream.hls import HLSStream
+
+log = logging.getLogger(__name__)
+
+
+@pluginmatcher(re.compile(r"https?://(?:www\.)?mixcloud\.com/live/(?P<user>[^/]+)"))
+class Mixcloud(Plugin):
+ def _get_streams(self):
+ data = self.session.http.post(
+ "https://www.mixcloud.com/graphql",
+ json={
+ "query": """
+ query streamData($user: UserLookup!) {
+ userLookup(lookup: $user) {
+ id
+ displayName
+ liveStream(isPublic: false) {
+ name
+ streamStatus
+ hlsUrl
+ }
+ }
+ }
+ """,
+ "variables": {"user": {"username": self.match.group("user")}}
+ },
+ schema=validate.Schema(
+ validate.parse_json(),
+ {
+ "data": {
+ "userLookup": validate.none_or_all(
+ {
+ "id": str,
+ "displayName": str,
+ "liveStream": {
+ "name": str,
+ "streamStatus": validate.any("ENDED", "LIVE"),
+ "hlsUrl": validate.none_or_all(validate.url()),
+ },
+ },
+ ),
+ },
+ },
+ validate.get(("data", "userLookup")),
+ ),
+ )
+ if not data:
+ log.error("User not found")
+ return
+
+ self.id = data.get("id")
+ self.author = data.get("displayName")
+ data = data.get("liveStream")
+
+ if data.get("streamStatus") == "ENDED":
+ log.info("This stream has ended")
+ return
+
+ self.title = data.get("name")
+
+ if data.get("hlsUrl"):
+ return HLSStream.parse_variant_playlist(self.session, data.get("hlsUrl"))
+
+
+__plugin__ = Mixcloud
| diff --git a/tests/plugins/test_mixcloud.py b/tests/plugins/test_mixcloud.py
new file mode 100644
--- /dev/null
+++ b/tests/plugins/test_mixcloud.py
@@ -0,0 +1,21 @@
+from streamlink.plugins.mixcloud import Mixcloud
+from tests.plugins import PluginCanHandleUrl
+
+
+class TestPluginCanHandleUrlMixcloud(PluginCanHandleUrl):
+ __plugin__ = Mixcloud
+
+ should_match_groups = [
+ ("http://mixcloud.com/live/user", {"user": "user"}),
+ ("http://www.mixcloud.com/live/user", {"user": "user"}),
+ ("https://mixcloud.com/live/user", {"user": "user"}),
+ ("https://www.mixcloud.com/live/user", {"user": "user"}),
+ ("https://www.mixcloud.com/live/user/anything", {"user": "user"}),
+ ]
+
+ should_not_match = [
+ "https://www.mixcloud.com/",
+ "https://www.mixcloud.com/live",
+ "https://www.mixcloud.com/live/",
+ "https://www.mixcloud.com/other",
+ ]
| Mixcloud Live
### Checklist
- [X] This is a plugin request and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin requests](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+request%22)
### Description
Mixcloud.com is a website that allows DJs and other musical events to upload and store DJ sets legally. They also provide an HLS based livestreaming feature that allows for the live broadcasting of video sets. These livestreams are what I am requesting support for.
There are no login checks, and I do not know of any region checks.
Thank you!
### Input URLs
https://www.mixcloud.com/live/Dj_Bridge/
https://www.mixcloud.com/live/AnthroNorthwest/
| 2023-01-16T22:43:01 |
|
streamlink/streamlink | 5,097 | streamlink__streamlink-5097 | [
"5094"
] | 91bf178e3868a91b714cc9066fb24d96f9ea79d6 | diff --git a/src/streamlink/plugins/tvp.py b/src/streamlink/plugins/tvp.py
--- a/src/streamlink/plugins/tvp.py
+++ b/src/streamlink/plugins/tvp.py
@@ -1,6 +1,7 @@
"""
$description Live TV channels and VODs from TVP, a Polish public, state-owned broadcaster.
-$url stream.tvp.pl
+$url tvp.info
+$url tvp.pl
$type live, vod
$notes Some VODs may be geo-restricted. Authentication is not supported.
"""
@@ -19,6 +20,7 @@
log = logging.getLogger(__name__)
+@pluginmatcher(re.compile(r"""https?://(?:www\.)?tvp\.info/"""), name="tvp_info")
@pluginmatcher(re.compile(
r"""
https?://
@@ -42,7 +44,7 @@ def _get_video_id(self, channel_id: Optional[str]):
"Connection": "close",
},
schema=validate.Schema(
- re.compile(r"window\.__channels\s*=\s*(?P<json>\[.+?])\s*;", re.DOTALL),
+ validate.regex(re.compile(r"window\.__channels\s*=\s*(?P<json>\[.+?])\s*;", re.DOTALL)),
validate.none_or_all(
validate.get("json"),
validate.parse_json(),
@@ -91,7 +93,7 @@ def _get_live(self, channel_id: Optional[str]):
"Referer": self.url,
},
schema=validate.Schema(
- re.compile(r"window\.__api__\s*=\s*(?P<json>\{.+?})\s*;", re.DOTALL),
+ validate.regex(re.compile(r"window\.__api__\s*=\s*(?P<json>\{.+?})\s*;", re.DOTALL)),
validate.get("json"),
validate.parse_json(),
{
@@ -169,8 +171,58 @@ def _get_vod(self, vod_id):
if data.get("HLS"):
return HLSStream.parse_variant_playlist(self.session, data["HLS"][0]["src"])
+ def _get_tvp_info_vod(self):
+ data = self.session.http.get(
+ self.url,
+ schema=validate.Schema(
+ validate.parse_html(),
+ validate.xml_xpath_string(".//script[contains(text(), 'window.__videoData')][1]/text()"),
+ validate.none_or_all(
+ str,
+ validate.regex(re.compile(r"window\.__videoData\s*=\s*(?P<json>{.*?})\s*;", re.DOTALL)),
+ validate.get("json"),
+ validate.parse_json(),
+ {
+ "_id": int,
+ "title": str,
+ },
+ ),
+ ),
+ )
+ if not data:
+ return
+
+ self.id = data.get("_id")
+ self.title = data.get("title")
+
+ data = self.session.http.get(
+ f"https://api.tvp.pl/tokenizer/token/{self.id}",
+ schema=validate.Schema(
+ validate.parse_json(),
+ {
+ "status": "OK",
+ "isGeoBlocked": bool,
+ "formats": [{
+ "mimeType": str,
+ "url": validate.url(),
+ }],
+ },
+ ),
+ )
+ log.debug(f"data={data}")
+
+ if data.get("isGeoBlocked"):
+ log.error("The content is not available in your region")
+ return
+
+ for format in data.get("formats"):
+ if format.get("mimeType") == "application/x-mpegurl":
+ return HLSStream.parse_variant_playlist(self.session, format.get("url"))
+
def _get_streams(self):
- if self.match["vod_id"]:
+ if self.matches["tvp_info"]:
+ return self._get_tvp_info_vod()
+ elif self.match["vod_id"]:
return self._get_vod(self.match["vod_id"])
else:
return self._get_live(self.match["channel_id"])
| diff --git a/tests/plugins/test_tvp.py b/tests/plugins/test_tvp.py
--- a/tests/plugins/test_tvp.py
+++ b/tests/plugins/test_tvp.py
@@ -27,6 +27,11 @@ class TestPluginCanHandleUrlTVP(PluginCanHandleUrl):
"https://vod.tvp.pl/programy,88/z-davidem-attenborough-dokola-swiata-odcinki,284703/odcinek-2,S01E02,319220",
{"vod_id": "319220"},
),
+
+ # tvp.info
+ ("https://tvp.info/", {}),
+ ("https://www.tvp.info/", {}),
+ ("https://www.tvp.info/65275202/13012023-0823", {}),
]
should_not_match = [
| tvp.info
### Checklist
- [X] This is a plugin request and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin requests](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+request%22)
### Description
existing tvp.py plugin supports vod.tvp.pl and some of tvp.info materials except one.
for example there are some daily materials which are not supported by this plugin.
### Input URLs
streamlink https://www.tvp.info/33822794/program-minela-8
streamlink https://www.tvp.info/65275202/13012023-0823
| 2023-01-17T15:19:48 |
|
streamlink/streamlink | 5,110 | streamlink__streamlink-5110 | [
"5045"
] | 90c1860580b634d15190242a79b4a8ad76509f76 | diff --git a/src/streamlink/plugins/earthcam.py b/src/streamlink/plugins/earthcam.py
--- a/src/streamlink/plugins/earthcam.py
+++ b/src/streamlink/plugins/earthcam.py
@@ -7,6 +7,7 @@
import logging
import re
+from urllib.parse import urlparse
from streamlink.plugin import Plugin, pluginmatcher
from streamlink.plugin.api import validate
@@ -45,7 +46,7 @@ def _get_streams(self):
if not data:
return
- cam_name = parse_qsd(self.url).get("cam") or next(iter(data.keys()), None)
+ cam_name = parse_qsd(urlparse(self.url).query).get("cam") or next(iter(data.keys()), None)
cam_data = data.get(cam_name)
if not cam_data:
return
| plugins.Earthcam: Not retrieving the "cam" argument. Always plays the first Cam. Fix included
### Checklist
- [X] This is a plugin issue and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest stable release
### Description
When a link contains a "cam" argument and the json has multiple cam entries, the plugin chooses the first one.
I changed the following lines that retrieve the cam argument from the url
```
parsed = urlparse(self.url)
cam_name = parse_qsd(parsed.query).get("cam") or next(iter(data.keys()), None)
```
also added the ```from urllib.parse import urlparse``` on top
And with those changes it works. The debug below is after the changes that I made
```streamlink -l debug "https://www.earthcam.com/events/christmascams/?cam=stpetersburg_xmas"```
### Debug log
```text
[cli][debug] OS: Linux-5.4.0-135-generic-x86_64-with-glibc2.29
[cli][debug] Python: 3.8.10
[cli][debug] Streamlink: 5.1.2
[cli][debug] Dependencies:
[cli][debug] certifi: 2021.5.30
[cli][debug] isodate: 0.6.0
[cli][debug] lxml: 4.9.2
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.11.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.26.0
[cli][debug] urllib3: 1.26.13
[cli][debug] websocket-client: 1.2.1
[cli][debug] importlib-metadata: 1.5.0
[cli][debug] Arguments:
[cli][debug] url=https://www.earthcam.com/events/christmascams/?cam=stpetersburg_xmas
[cli][debug] --loglevel=debug
[cli][info] Found matching plugin earthcam for URL https://www.earthcam.com/events/christmascams/?cam=stpetersburg_xmas
[plugins.earthcam][debug] Found cam stpetersburg_xmas
```
| 2023-01-22T01:00:52 |
||
streamlink/streamlink | 5,137 | streamlink__streamlink-5137 | [
"5136"
] | fbb9ed649757c53b2b1b135cd40cbb9d0ac41e7a | diff --git a/src/streamlink/plugins/youtube.py b/src/streamlink/plugins/youtube.py
--- a/src/streamlink/plugins/youtube.py
+++ b/src/streamlink/plugins/youtube.py
@@ -22,26 +22,18 @@
log = logging.getLogger(__name__)
-@pluginmatcher(re.compile(r"""
- https?://(?:\w+\.)?youtube\.com/
- (?:
- (?:
- (?:
- watch\?(?:.*&)*v=
- |
- (?P<embed>embed)/(?!live_stream)
- |
- v/
- )(?P<video_id>[\w-]{11})
- )
- |
- embed/live_stream\?channel=(?P<embed_live>[^/?&]+)
- |
- (?:c(?:hannel)?/|user/)?(?P<channel>[^/?]+)(?P<channel_live>/live)?/?$
- )
- |
- https?://youtu\.be/(?P<video_id_short>[\w-]{11})
-""", re.VERBOSE))
+@pluginmatcher(name="default", pattern=re.compile(
+ r"https?://(?:\w+\.)?youtube\.com/(?:v/|live/|watch\?(?:.*&)?v=)(?P<video_id>[\w-]{11})",
+))
+@pluginmatcher(name="channel", pattern=re.compile(
+ r"https?://(?:\w+\.)?youtube\.com/(?:@|c(?:hannel)?/)?(?P<channel>[^/?]+)(?P<live>/live)?/?$",
+))
+@pluginmatcher(name="embed", pattern=re.compile(
+ r"https?://(?:\w+\.)?youtube\.com/embed/(?:live_stream\?channel=(?P<live>[^/?&]+)|(?P<video_id>[\w-]{11}))",
+))
+@pluginmatcher(name="shorthand", pattern=re.compile(
+ r"https?://youtu\.be/(?P<video_id>[\w-]{11})",
+))
class YouTube(Plugin):
_re_ytInitialData = re.compile(r"""var\s+ytInitialData\s*=\s*({.*?})\s*;\s*</script>""", re.DOTALL)
_re_ytInitialPlayerResponse = re.compile(r"""var\s+ytInitialPlayerResponse\s*=\s*({.*?});\s*var\s+\w+\s*=""", re.DOTALL)
@@ -81,12 +73,12 @@ def __init__(self, *args, **kwargs):
# translate input URLs to be able to find embedded data and to avoid unnecessary HTTP redirects
if parsed.netloc == "gaming.youtube.com":
self.url = urlunparse(parsed._replace(scheme="https", netloc="www.youtube.com"))
- elif self.match.group("video_id_short") is not None:
- self.url = self._url_canonical.format(video_id=self.match.group("video_id_short"))
- elif self.match.group("embed") is not None:
- self.url = self._url_canonical.format(video_id=self.match.group("video_id"))
- elif self.match.group("embed_live") is not None:
- self.url = self._url_channelid_live.format(channel_id=self.match.group("embed_live"))
+ elif self.matches["shorthand"]:
+ self.url = self._url_canonical.format(video_id=self.match["video_id"])
+ elif self.matches["embed"] and self.match["video_id"]:
+ self.url = self._url_canonical.format(video_id=self.match["video_id"])
+ elif self.matches["embed"] and self.match["live"]:
+ self.url = self._url_channelid_live.format(channel_id=self.match["live"])
elif parsed.scheme != "https":
self.url = urlunparse(parsed._replace(scheme="https"))
@@ -132,7 +124,7 @@ def _schema_canonical(self, data):
schema_canonical = validate.Schema(
validate.parse_html(),
validate.xml_xpath_string(".//link[@rel='canonical'][1]/@href"),
- validate.transform(self.matcher.match),
+ validate.regex(self.matchers["default"].pattern),
validate.get("video_id")
)
return schema_canonical.validate(data)
@@ -281,7 +273,11 @@ def _get_data_from_regex(res, regex, descr):
return parse_json(match.group(1))
def _get_data_from_api(self, res):
- _i_video_id = self.match.group("video_id")
+ try:
+ _i_video_id = self.match["video_id"]
+ except IndexError:
+ _i_video_id = None
+
if _i_video_id is None:
try:
_i_video_id = self._schema_canonical(res.text)
@@ -343,7 +339,7 @@ def _data_status(self, data, errorlog=False):
def _get_streams(self):
res = self._get_res(self.url)
- if self.match.group("channel") and not self.match.group("channel_live"):
+ if self.matches["channel"] and not self.match["live"]:
initial = self._get_data_from_regex(res, self._re_ytInitialData, "initial data")
video_id = self._data_video_id(initial)
if video_id is None:
| diff --git a/tests/plugins/test_youtube.py b/tests/plugins/test_youtube.py
--- a/tests/plugins/test_youtube.py
+++ b/tests/plugins/test_youtube.py
@@ -9,40 +9,54 @@
class TestPluginCanHandleUrlYouTube(PluginCanHandleUrl):
__plugin__ = YouTube
- should_match = [
- "https://www.youtube.com/CHANNELNAME",
- "https://www.youtube.com/CHANNELNAME/",
- "https://www.youtube.com/CHANNELNAME/live",
- "https://www.youtube.com/CHANNELNAME/live/",
- "https://www.youtube.com/c/CHANNELNAME",
- "https://www.youtube.com/c/CHANNELNAME/",
- "https://www.youtube.com/c/CHANNELNAME/live",
- "https://www.youtube.com/c/CHANNELNAME/live/",
- "https://www.youtube.com/user/CHANNELNAME",
- "https://www.youtube.com/user/CHANNELNAME/",
- "https://www.youtube.com/user/CHANNELNAME/live",
- "https://www.youtube.com/user/CHANNELNAME/live/",
- "https://www.youtube.com/channel/CHANNELID",
- "https://www.youtube.com/channel/CHANNELID/",
- "https://www.youtube.com/channel/CHANNELID/live",
- "https://www.youtube.com/channel/CHANNELID/live/",
- "https://www.youtube.com/embed/aqz-KE-bpKQ",
- "https://www.youtube.com/embed/live_stream?channel=UCNye-wNBqNL5ZzHSJj3l8Bg",
- "https://www.youtube.com/v/aqz-KE-bpKQ",
- "https://www.youtube.com/watch?v=aqz-KE-bpKQ",
- "https://www.youtube.com/watch?foo=bar&baz=qux&v=aqz-KE-bpKQ",
- "https://youtu.be/0123456789A",
- ]
-
should_match_groups = [
- ("https://www.youtube.com/v/aqz-KE-bpKQ", {
+ (("default", "https://www.youtube.com/v/aqz-KE-bpKQ"), {
"video_id": "aqz-KE-bpKQ",
}),
- ("https://www.youtube.com/embed/aqz-KE-bpKQ", {
- "embed": "embed",
+ (("default", "https://www.youtube.com/live/aqz-KE-bpKQ"), {
+ "video_id": "aqz-KE-bpKQ",
+ }),
+ (("default", "https://www.youtube.com/watch?foo=bar&baz=qux&v=aqz-KE-bpKQ&asdf=1234"), {
+ "video_id": "aqz-KE-bpKQ",
+ }),
+
+ (("channel", "https://www.youtube.com/CHANNELNAME"), {
+ "channel": "CHANNELNAME",
+ }),
+ (("channel", "https://www.youtube.com/CHANNELNAME/live"), {
+ "channel": "CHANNELNAME",
+ "live": "/live",
+ }),
+ (("channel", "https://www.youtube.com/@CHANNELNAME"), {
+ "channel": "CHANNELNAME",
+ }),
+ (("channel", "https://www.youtube.com/@CHANNELNAME/live"), {
+ "channel": "CHANNELNAME",
+ "live": "/live",
+ }),
+ (("channel", "https://www.youtube.com/c/CHANNELNAME"), {
+ "channel": "CHANNELNAME",
+ }),
+ (("channel", "https://www.youtube.com/c/CHANNELNAME/live"), {
+ "channel": "CHANNELNAME",
+ "live": "/live",
+ }),
+ (("channel", "https://www.youtube.com/channel/CHANNELID"), {
+ "channel": "CHANNELID",
+ }),
+ (("channel", "https://www.youtube.com/channel/CHANNELID/live"), {
+ "channel": "CHANNELID",
+ "live": "/live",
+ }),
+
+ (("embed", "https://www.youtube.com/embed/aqz-KE-bpKQ"), {
"video_id": "aqz-KE-bpKQ",
}),
- ("https://www.youtube.com/watch?v=aqz-KE-bpKQ", {
+ (("embed", "https://www.youtube.com/embed/live_stream?channel=CHANNELNAME"), {
+ "live": "CHANNELNAME",
+ }),
+
+ (("shorthand", "https://youtu.be/aqz-KE-bpKQ"), {
"video_id": "aqz-KE-bpKQ",
}),
]
| plugins.youtube: New URL formats are unsupported
### Checklist
- [X] This is a plugin issue and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest build from the master branch
### Description
Trying to open these URL formats lead to same `error: No plugin can handle URL` error.
Affected URL formats are at least:
```
https://www.youtube.com/live/<id>'
https://www.youtube.com/@<handle>/streams
```
### Debug log
```text
$ streamlink -l debug https://www.youtube.com/live/21X5lGlDOfg
[cli][debug] OS: Linux-5.15.0-58-generic-x86_64-with-glibc2.35
[cli][debug] Python: 3.10.6
[cli][debug] Streamlink: 5.2.1+7.gfbb9ed64
[cli][debug] Dependencies:
[cli][debug] certifi: 2022.6.15
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.8.0
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.15.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.28.1
[cli][debug] urllib3: 1.26.5
[cli][debug] websocket-client: 1.3.3
[cli][debug] importlib-metadata: 4.6.4
[cli][debug] Arguments:
[cli][debug] url=https://www.youtube.com/live/21X5lGlDOfg
[cli][debug] --loglevel=debug
[cli][debug] --player=mpv --cache
[cli][debug] --default-stream=['best']
error: No plugin can handle URL: https://www.youtube.com/live/21X5lGlDOfg
```
| 2023-02-01T17:58:21 |
|
streamlink/streamlink | 5,147 | streamlink__streamlink-5147 | [
"5114"
] | 04da9f5cdcf06d44618c0547d14f7f3dae6db1cd | diff --git a/src/streamlink/plugins/nimotv.py b/src/streamlink/plugins/nimotv.py
--- a/src/streamlink/plugins/nimotv.py
+++ b/src/streamlink/plugins/nimotv.py
@@ -9,7 +9,7 @@
from streamlink.plugin import Plugin, pluginmatcher
from streamlink.plugin.api import useragents, validate
-from streamlink.stream.hls import HLSStream
+from streamlink.stream.http import HTTPStream
log = logging.getLogger(__name__)
@@ -32,6 +32,8 @@ class NimoTV(Plugin):
_re_domain = re.compile(br'(https?:\/\/[A-Za-z]{2,3}.hls[A-Za-z\.\/]+)(?:V|&)')
_re_id = re.compile(br'id=([^|\\]+)')
_re_tp = re.compile(br'tp=(\d+)')
+ _re_wsSecret = re.compile(br'wsSecret=(\w+)')
+ _re_wsTime = re.compile(br'wsTime=(\w+)')
def _get_streams(self):
username = self.match.group('username')
@@ -74,6 +76,8 @@ def _get_streams(self):
_domain = self._re_domain.search(mStreamPkg).group(1).decode('utf-8')
_id = self._re_id.search(mStreamPkg).group(1).decode('utf-8')
_tp = self._re_tp.search(mStreamPkg).group(1).decode('utf-8')
+ _wsSecret = self._re_wsSecret.search(mStreamPkg).group(1).decode('utf-8')
+ _wsTime = self._re_wsTime.search(mStreamPkg).group(1).decode('utf-8')
except AttributeError:
log.error('invalid mStreamPkg')
return
@@ -82,11 +86,14 @@ def _get_streams(self):
'appid': _appid,
'id': _id,
'tp': _tp,
+ 'wsSecret': _wsSecret,
+ 'wsTime': _wsTime,
'u': '0',
't': '100',
'needwm': 1,
}
- url = f'{_domain}{_id}.m3u8'
+ url = f'{_domain}{_id}.flv'
+ url = url.replace('hls.nimo.tv', 'flv.nimo.tv')
log.debug(f'URL={url}')
for k, v in self.video_qualities.items():
_params = params.copy()
@@ -98,7 +105,7 @@ def _get_streams(self):
log.trace(f'{v} params={_params!r}')
# some qualities might not exist, but it will select a different lower quality
- yield v, HLSStream(self.session, url, params=_params)
+ yield v, HTTPStream(self.session, url, params=_params)
self.author = data['nickname']
self.category = data['game']
| plugins.nimotv: live stream stops after couple of seconds
### Checklist
- [X] This is a plugin issue and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest stable release
### Description
try any live streams of nimo.tv, the live stream will stop in couple of seconds.
### Debug log
```text
c:\temp>streamlink --loglevel debug https://www.nimo.tv/live/97747608 best
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.7.8
[cli][debug] Streamlink: 5.1.2
[cli][debug] Dependencies:
[cli][debug] certifi: 2018.11.29
[cli][debug] isodate: 0.6.0
[cli][debug] lxml: 4.6.4
[cli][debug] pycountry: 20.7.3
[cli][debug] pycryptodome: 3.7.3
[cli][debug] PySocks: 1.6.8
[cli][debug] requests: 2.26.0
[cli][debug] urllib3: 1.26.12
[cli][debug] websocket-client: 1.2.1
[cli][debug] importlib-metadata: 3.10.0
[cli][debug] Arguments:
[cli][debug] url=https://www.nimo.tv/live/97747608
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][info] Found matching plugin nimotv for URL https://www.nimo.tv/live/97747608
[plugins.nimotv][debug] URL=http://tx.hls.nimo.tv/live/su1629530778249rea7ea30592d8ab7ce78a1a13e3037be7.m3u8
[cli][info] Available streams: 240p (worst), 360p, 480p, 720p, 1080p (best)
[cli][info] Opening stream: 1080p (hls)
[cli][info] Starting player: "C:\Program Files\VideoLAN\VLC\vlc.exe"
[stream.hls][debug] Reloading playlist
[cli][debug] Pre-buffering 8192 bytes
[stream.hls][debug] First Sequence: 1674443953; Last Sequence: 1674443955
[stream.hls][debug] Start offset: 0; Duration: None; Start Sequence: 1674443953; End Sequence: None
[stream.hls][debug] Adding segment 1674443953 to queue
[stream.hls][debug] Adding segment 1674443954 to queue
[stream.hls][debug] Adding segment 1674443955 to queue
[stream.hls][debug] Writing segment 1674443953 to output
[stream.hls][debug] Segment 1674443953 complete
[cli.output][debug] Opening subprocess: "C:\Program Files\VideoLAN\VLC\vlc.exe" --input-title-format https://www.nimo.tv/live/97747608 -
[cli][debug] Writing stream to output
[stream.hls][debug] Writing segment 1674443954 to output
[stream.hls][debug] Segment 1674443954 complete
[stream.hls][debug] Writing segment 1674443955 to output
[stream.hls][debug] Segment 1674443955 complete
[stream.hls][debug] Reloading playlist
[stream.hls][warning] Failed to reload playlist: Unable to open URL: http://tx.hls.nimo.tv/live/su1629530778249rea7ea30592d8ab7ce78a1a13e3037be7.m3u8?appid=81&id=su1629530778249rea7ea30592d8ab7ce78a1a13e3037be7&tp=1674443951824&u=0&t=100&needwm=0&ratio=6000 (403 Client Error: Forbidden for url: http://tx.hls.nimo.tv/live/su1629530778249rea7ea30592d8ab7ce78a1a13e3037be7.m3u8?appid=81&id=su1629530778249rea7ea30592d8ab7ce78a1a13e3037be7&tp=1674443951824&u=0&t=100&needwm=0&ratio=6000&appid=81&id=su1629530778249rea7ea30592d8ab7ce78a1a13e3037be7&tp=1674443951824&u=0&t=100&needwm=0&ratio=6000)
[stream.hls][debug] Reloading playlist
[stream.hls][warning] Failed to reload playlist: Unable to open URL: http://tx.hls.nimo.tv/live/su1629530778249rea7ea30592d8ab7ce78a1a13e3037be7.m3u8?appid=81&id=su1629530778249rea7ea30592d8ab7ce78a1a13e3037be7&tp=1674443951824&u=0&t=100&needwm=0&ratio=6000 (403 Client Error: Forbidden for url: http://tx.hls.nimo.tv/live/su1629530778249rea7ea30592d8ab7ce78a1a13e3037be7.m3u8?appid=81&id=su1629530778249rea7ea30592d8ab7ce78a1a13e3037be7&tp=1674443951824&u=0&t=100&needwm=0&ratio=6000&appid=81&id=su1629530778249rea7ea30592d8ab7ce78a1a13e3037be7&tp=1674443951824&u=0&t=100&needwm=0&ratio=6000)
[stream.hls][debug] Reloading playlist
[stream.hls][warning] Failed to reload playlist: Unable to open URL: http://tx.hls.nimo.tv/live/su1629530778249rea7ea30592d8ab7ce78a1a13e3037be7.m3u8?appid=81&id=su1629530778249rea7ea30592d8ab7ce78a1a13e3037be7&tp=1674443951824&u=0&t=100&needwm=0&ratio=6000 (403 Client Error: Forbidden for url: http://tx.hls.nimo.tv/live/su1629530778249rea7ea30592d8ab7ce78a1a13e3037be7.m3u8?appid=81&id=su1629530778249rea7ea30592d8ab7ce78a1a13e3037be7&tp=1674443951824&u=0&t=100&needwm=0&ratio=6000&appid=81&id=su1629530778249rea7ea30592d8ab7ce78a1a13e3037be7&tp=1674443951824&u=0&t=100&needwm=0&ratio=6000)
```
| 2023-02-08T13:54:15 |
||
streamlink/streamlink | 5,162 | streamlink__streamlink-5162 | [
"5159"
] | 76480b0dec1773eeea9810e67317dcd87e152cd4 | diff --git a/src/streamlink/stream/ffmpegmux.py b/src/streamlink/stream/ffmpegmux.py
--- a/src/streamlink/stream/ffmpegmux.py
+++ b/src/streamlink/stream/ffmpegmux.py
@@ -4,6 +4,7 @@
import subprocess
import sys
import threading
+from contextlib import suppress
from functools import lru_cache
from pathlib import Path
from shutil import which
@@ -131,22 +132,28 @@ def _resolve_command(cls, command: Optional[str] = None, validate: bool = True)
@staticmethod
def copy_to_pipe(stream: StreamIO, pipe: NamedPipeBase):
log.debug(f"Starting copy to pipe: {pipe.path}")
+ # TODO: catch OSError when creating/opening pipe fails and close entire output stream
pipe.open()
- while not stream.closed:
+
+ while True:
try:
data = stream.read(8192)
- if len(data):
- pipe.write(data)
- else:
- break
- except (OSError, ValueError):
- log.error(f"Pipe copy aborted: {pipe.path}")
+ except (OSError, ValueError) as err:
+ log.error(f"Error while reading from substream: {err}")
+ break
+
+ if data == b"":
+ log.debug(f"Pipe copy complete: {pipe.path}")
+ break
+
+ try:
+ pipe.write(data)
+ except OSError as err:
+ log.error(f"Error while writing to pipe {pipe.path}: {err}")
break
- try:
+
+ with suppress(OSError):
pipe.close()
- except OSError: # might fail closing, but that should be ok for the pipe
- pass
- log.debug(f"Pipe copy complete: {pipe.path}")
def __init__(self, session, *streams, **options):
if not self.is_usable(session):
@@ -223,17 +230,25 @@ def close(self):
self.process.kill()
self.process.stdout.close()
- # close the streams
executor = concurrent.futures.ThreadPoolExecutor()
+
+ # close the substreams
futures = [
executor.submit(stream.close)
for stream in self.streams
if hasattr(stream, "close") and callable(stream.close)
]
-
concurrent.futures.wait(futures, return_when=concurrent.futures.ALL_COMPLETED)
log.debug("Closed all the substreams")
+ # wait for substream copy-to-pipe threads to terminate and clean up the opened pipes
+ timeout = self.session.options.get("stream-timeout")
+ futures = [
+ executor.submit(thread.join, timeout=timeout)
+ for thread in self.pipe_threads
+ ]
+ concurrent.futures.wait(futures, return_when=concurrent.futures.ALL_COMPLETED)
+
if self.close_errorlog:
self.errorlog.close()
self.errorlog = None
diff --git a/src/streamlink/utils/named_pipe.py b/src/streamlink/utils/named_pipe.py
--- a/src/streamlink/utils/named_pipe.py
+++ b/src/streamlink/utils/named_pipe.py
@@ -4,6 +4,7 @@
import random
import tempfile
import threading
+from contextlib import suppress
from pathlib import Path
from streamlink.compat import is_win32
@@ -65,11 +66,15 @@ def write(self, data):
return self.fifo.write(data)
def close(self):
- if self.fifo:
- self.fifo.close()
+ try:
+ if self.fifo is not None:
+ self.fifo.close()
+ except OSError:
+ raise
+ finally:
+ with suppress(OSError):
+ self.path.unlink()
self.fifo = None
- if self.path.is_fifo():
- os.unlink(self.path)
class NamedPipeWindows(NamedPipeBase):
@@ -118,9 +123,13 @@ def write(self, data):
return written.value
def close(self):
- if self.pipe is not None:
- windll.kernel32.DisconnectNamedPipe(self.pipe)
- windll.kernel32.CloseHandle(self.pipe)
+ try:
+ if self.pipe is not None:
+ windll.kernel32.DisconnectNamedPipe(self.pipe)
+ windll.kernel32.CloseHandle(self.pipe)
+ except OSError:
+ raise
+ finally:
self.pipe = None
| stream.ffmpegmux: race condition when copying data to named pipe
### Checklist
- [X] This is a bug report and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed bug reports](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22bug%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest build from the master branch
### Description
Opening this issue myself, because the person who messaged me doesn't want to create a GitHub account...
After having a look at an [example DASH stream from Vimeo that was sent to me](https://vimeo.com/channels/staffpicks/790905324), there appears to be a race condition within `FFMPEGMuxer.copy_to_pipe()`. This is where the stream data of each substream gets written to a named pipe where the FFmpeg process then reads from and muxes the streams. The read-write loop ends as soon as the stream's `closed` attribute is set (by its stream implementation in another thread):
https://github.com/streamlink/streamlink/blame/a0a5af60b0e99f3ee7987b1caad12497884a4062/src/streamlink/stream/ffmpegmux.py#L135
While rewriting the CLI's `StreamRunner` recently, I initially had the same condition in its read-write loop and then figured that this would be a bad idea because the buffer sometimes wouldn't get emptied at the end of the stream when all segments have finished downloading for example. This seems to be the case here, too.
https://github.com/streamlink/streamlink/blame/a0a5af60b0e99f3ee7987b1caad12497884a4062/src/streamlink_cli/streamrunner.py#L126
The following diff should work, but I will have to take a closer look first. Opening this issue thread as a reminder to check later in more detail.
```diff
diff --git a/src/streamlink/stream/ffmpegmux.py b/src/streamlink/stream/ffmpegmux.py
index 29a928aa..f48a7668 100644
--- a/src/streamlink/stream/ffmpegmux.py
+++ b/src/streamlink/stream/ffmpegmux.py
@@ -132,14 +132,16 @@ class FFMPEGMuxer(StreamIO):
def copy_to_pipe(stream: StreamIO, pipe: NamedPipeBase):
log.debug(f"Starting copy to pipe: {pipe.path}")
pipe.open()
- while not stream.closed:
+ while True:
try:
data = stream.read(8192)
- if len(data):
- pipe.write(data)
- else:
+ if data == b"":
break
except (OSError, ValueError):
+ break
+ try:
+ pipe.write(data)
+ except OSError:
log.error(f"Pipe copy aborted: {pipe.path}")
break
try:
```
### Debug log
```text
$ streamlink -l debug -o /tmp/foo.mkv 'https://vimeo.com/channels/staffpicks/790905324' best
...
[stream.dash][debug] Download of segment: 0c433f4a.mp4 complete
[stream.dash][debug] Download of segment: 9ae75d32.mp4 complete
...
[stream.dash][debug] Download of segment: 0c433f4a.mp4 complete
[stream.dash][debug] Download of segment: 9ae75d32.mp4 complete
[stream.segmented][debug] Closing writer thread
[stream.ffmpegmux][debug] Pipe copy complete: /tmp/streamlinkpipe-812266-2-8643
[stream.dash][debug] Download of segment: 0c433f4a.mp4 complete
[stream.dash][debug] Download of segment: 0c433f4a.mp4 complete
...
[stream.dash][debug] Download of segment: 0c433f4a.mp4 complete
[stream.dash][debug] Download of segment: 0c433f4a.mp4 complete
[stream.segmented][debug] Closing writer thread
[stream.ffmpegmux][debug] Pipe copy complete: /tmp/streamlinkpipe-812266-1-2639
[stream.ffmpegmux][debug] Closing ffmpeg thread
[stream.ffmpegmux][debug] Closed all the substreams
[cli][info] Stream ended
[cli][info] Closing currently open stream...
$ ffmpeg -i /tmp/foo.mkv -c copy /tmp/foo.mp4
...
$ ffprobe -v error -of json -show_streams /tmp/foo.mp4 | jq -r .streams[].duration
906.832313
725.887667
```
| The proposed patch should be good enough. I will have another look at this tomorrow, so this specific issue can get fixed at least.
After that, I'll give `FFMPEGMuxer` a proper rewrite, because it's really messy, has lots of other issues, and it doesn't have any tests apart from the is-usable stuff and options setup. Related: #4998 | 2023-02-14T19:10:20 |
|
streamlink/streamlink | 5,189 | streamlink__streamlink-5189 | [
"4215"
] | 65bb38e42e58b030c37c581d78fb90023b928647 | diff --git a/src/streamlink/stream/dash_manifest.py b/src/streamlink/stream/dash_manifest.py
--- a/src/streamlink/stream/dash_manifest.py
+++ b/src/streamlink/stream/dash_manifest.py
@@ -356,6 +356,10 @@ class Initialization(MPDNode):
def __init__(self, node, root=None, parent=None, *args, **kwargs):
super().__init__(node, root, parent, *args, **kwargs)
self.source_url = self.attr("sourceURL")
+ self.range = self.attr(
+ "range",
+ parser=MPDParsers.range,
+ )
class SegmentURL(MPDNode):
@@ -407,6 +411,7 @@ def segments(self):
duration=0,
init=True,
content=False,
+ byterange=self.initialization.range,
)
for n, segment_url in enumerate(self.segment_urls, self.start_number):
yield Segment(
| diff --git a/tests/resources/dash/test_segments_byterange.mpd b/tests/resources/dash/test_segments_byterange.mpd
new file mode 100644
--- /dev/null
+++ b/tests/resources/dash/test_segments_byterange.mpd
@@ -0,0 +1,33 @@
+<?xml version="1.0"?>
+<MPD
+ xmlns="urn:mpeg:dash:schema:mpd:2011"
+ profiles="urn:mpeg:dash:profile:isoff-live:2011"
+ minBufferTime="PT10.01S"
+ mediaPresentationDuration="PT30.097S"
+ type="static"
+>
+ <Period>
+ <AdaptationSet mimeType="video/mp4" segmentAlignment="true" startWithSAP="1" maxWidth="768" maxHeight="432">
+ <Representation id="video-avc1" codecs="avc1.4D401E" width="768" height="432" scanType="progressive" frameRate="30000/1001" bandwidth="699597">
+ <SegmentList timescale="1000" duration="10010">
+ <Initialization sourceURL="video-frag.mp4" range="36-746"/>
+ <SegmentURL media="video-frag.mp4" mediaRange="747-876117"/>
+ <SegmentURL media="video-frag.mp4" mediaRange="876118-1466913"/>
+ <SegmentURL media="video-frag.mp4" mediaRange="1466914-1953954"/>
+ <SegmentURL media="video-frag.mp4" mediaRange="1953955-1994652"/>
+ </SegmentList>
+ </Representation>
+ </AdaptationSet>
+ <AdaptationSet mimeType="audio/mp4" startWithSAP="1" segmentAlignment="true">
+ <Representation id="audio-und-mp4a.40.2" codecs="mp4a.40.2" bandwidth="98808" audioSamplingRate="48000">
+ <SegmentList timescale="1000" duration="10010">
+ <Initialization sourceURL="audio-frag.mp4" range="32-623"/>
+ <SegmentURL media="audio-frag.mp4" mediaRange="624-124199"/>
+ <SegmentURL media="audio-frag.mp4" mediaRange="124200-250303"/>
+ <SegmentURL media="audio-frag.mp4" mediaRange="250304-374365"/>
+ <SegmentURL media="audio-frag.mp4" mediaRange="374366-374836"/>
+ </SegmentList>
+ </Representation>
+ </AdaptationSet>
+ </Period>
+</MPD>
diff --git a/tests/stream/test_dash_parser.py b/tests/stream/test_dash_parser.py
--- a/tests/stream/test_dash_parser.py
+++ b/tests/stream/test_dash_parser.py
@@ -261,3 +261,29 @@ def test_segments_static_periods_duration(self):
mpd = MPD(mpd_xml, base_url="http://test.se/", url="http://test.se/manifest.mpd")
duration = mpd.periods[0].duration.total_seconds()
assert duration == pytest.approx(204.32)
+
+ def test_segments_byterange(self):
+ with xml("dash/test_segments_byterange.mpd") as mpd_xml:
+ mpd = MPD(mpd_xml, base_url="http://test/", url="http://test/manifest.mpd")
+ assert [
+ [
+ (seg.url, seg.init, seg.byterange)
+ for seg in adaptationset.representations[0].segments()
+ ]
+ for adaptationset in mpd.periods[0].adaptationSets
+ ] == [
+ [
+ ("http://test/video-frag.mp4", True, (36, 711)),
+ ("http://test/video-frag.mp4", False, (747, 875371)),
+ ("http://test/video-frag.mp4", False, (876118, 590796)),
+ ("http://test/video-frag.mp4", False, (1466914, 487041)),
+ ("http://test/video-frag.mp4", False, (1953955, 40698)),
+ ],
+ [
+ ("http://test/audio-frag.mp4", True, (32, 592)),
+ ("http://test/audio-frag.mp4", False, (624, 123576)),
+ ("http://test/audio-frag.mp4", False, (124200, 126104)),
+ ("http://test/audio-frag.mp4", False, (250304, 124062)),
+ ("http://test/audio-frag.mp4", False, (374366, 471)),
+ ],
+ ]
| stream.dash: range attribute in initialization segment
### Checklist
- [X] This is a bug report and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed bug reports](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22bug%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest stable release
### Description
It seems that `dash_manifest.py` needs to support range attribute of initialization segment.
I played mpd made with Bento4 tools: `mp4dash -f -o bento4 --mpd-name=manifest.mpd --no-split --use-segment-list video-frag.mp4 audio-frag.mp4`
Video and audio play normally once, but it won't stop there. Playback continues, video stops motion with errors and audio plays.
This is the content of mpd:
```
<?xml version="1.0" ?>
<MPD xmlns="urn:mpeg:dash:schema:mpd:2011" profiles="urn:mpeg:dash:profile:isoff-live:2011" minBufferTime="PT10.01S" mediaPresentationDuration="PT30.097S" type="static">
<!-- Created with Bento4 mp4-dash.py, VERSION=2.0.0-639 -->
<Period>
<!-- Video -->
<AdaptationSet mimeType="video/mp4" segmentAlignment="true" startWithSAP="1" maxWidth="768" maxHeight="432">
<Representation id="video-avc1" codecs="avc1.4D401E" width="768" height="432" scanType="progressive" frameRate="30000/1001" bandwidth="699597">
<SegmentList timescale="1000" duration="10010">
<Initialization sourceURL="video-frag.mp4" range="36-746"/>
<SegmentURL media="video-frag.mp4" mediaRange="747-876117"/>
<SegmentURL media="video-frag.mp4" mediaRange="876118-1466913"/>
<SegmentURL media="video-frag.mp4" mediaRange="1466914-1953954"/>
<SegmentURL media="video-frag.mp4" mediaRange="1953955-1994652"/>
</SegmentList>
</Representation>
</AdaptationSet>
<!-- Audio -->
<AdaptationSet mimeType="audio/mp4" startWithSAP="1" segmentAlignment="true">
<Representation id="audio-und-mp4a.40.2" codecs="mp4a.40.2" bandwidth="98808" audioSamplingRate="48000">
<AudioChannelConfiguration schemeIdUri="urn:mpeg:mpegB:cicp:ChannelConfiguration" value="2"/>
<SegmentList timescale="1000" duration="10010">
<Initialization sourceURL="audio-frag.mp4" range="32-623"/>
<SegmentURL media="audio-frag.mp4" mediaRange="624-124199"/>
<SegmentURL media="audio-frag.mp4" mediaRange="124200-250303"/>
<SegmentURL media="audio-frag.mp4" mediaRange="250304-374365"/>
<SegmentURL media="audio-frag.mp4" mediaRange="374366-374836"/>
</SegmentList>
</Representation>
</AdaptationSet>
</Period>
</MPD>
```
I tried this mpd directly with mpv: `mpv http://127.0.0.1:8888/bento4/manifest.mpd`
Warnings are reported many times (`[ffmpeg] Invalid return value 0 for stream protocol`), but video and audio seem to play fine, just once then stop.
### Debug log
```text
streamlink -l debug http://127.0.0.1:8888/bento4/manifest.mpd best
[cli][debug] OS: macOS 10.12.6
[cli][debug] Python: 3.9.9
[cli][debug] Streamlink: 3.0.2
[cli][debug] Requests(2.26.0), Socks(1.7.1), Websocket(1.2.1)
[cli][debug] Arguments:
[cli][debug] url=http://127.0.0.1:8888/bento4/manifest.mpd
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --player=mpv
[cli][debug] --verbose-player=True
[cli][debug] --player-no-close=True
[cli][info] Found matching plugin dash for URL http://127.0.0.1:8888/bento4/manifest.mpd
[plugins.dash][debug] Parsing MPD URL: http://127.0.0.1:8888/bento4/manifest.mpd
[utils.l10n][debug] Language code: en_US
[stream.dash][debug] Available languages for DASH audio streams: NONE (using: n/a)
[cli][info] Available streams: 432p (worst, best)
[cli][info] Opening stream: 432p (dash)
[stream.dash][debug] Opening DASH reader for: video-avc1 (video/mp4)
[stream.dash][debug] Opening DASH reader for: audio-und-mp4a.40.2 (audio/mp4)
[utils.named_pipe][info] Creating pipe streamlinkpipe-55925-1-9651
[utils.named_pipe][info] Creating pipe streamlinkpipe-55925-2-8564
[stream.ffmpegmux][debug] ffmpeg command: ffmpeg -nostats -y -i /var/tmp/streamlinkpipe-55925-1-9651 -i /var/tmp/streamlinkpipe-55925-2-8564 -c:v copy -c:a copy -copyts -f matroska pipe:1
[stream.ffmpegmux][debug] Starting copy to pipe: /var/tmp/streamlinkpipe-55925-1-9651
[stream.ffmpegmux][debug] Starting copy to pipe: /var/tmp/streamlinkpipe-55925-2-8564
[cli][debug] Pre-buffering 8192 bytes
[stream.dash][debug] Download of segment: http://127.0.0.1:8888/bento4/audio-frag.mp4 complete
[stream.dash][debug] Download of segment: http://127.0.0.1:8888/bento4/audio-frag.mp4 complete
[stream.dash][debug] Download of segment: http://127.0.0.1:8888/bento4/video-frag.mp4 complete
[stream.dash][debug] Download of segment: http://127.0.0.1:8888/bento4/audio-frag.mp4 complete
[cli][info] Starting player: mpv
[cli.output][debug] Opening subprocess: mpv --force-media-title=http://127.0.0.1:8888/bento4/manifest.mpd -
[stream.dash][debug] Download of segment: http://127.0.0.1:8888/bento4/audio-frag.mp4 complete
[stream.dash][debug] Download of segment: http://127.0.0.1:8888/bento4/video-frag.mp4 complete
[stream.dash][debug] Download of segment: http://127.0.0.1:8888/bento4/audio-frag.mp4 complete
[stream.dash][debug] Download of segment: http://127.0.0.1:8888/bento4/video-frag.mp4 complete
[stream.dash][debug] Download of segment: http://127.0.0.1:8888/bento4/video-frag.mp4 complete
[stream.dash][debug] Download of segment: http://127.0.0.1:8888/bento4/video-frag.mp4 complete
[file] Reading from stdin...
[cli][debug] Writing stream to output
(+) Video --vid=1 (*) (h264 768x432 29.970fps)
(+) Audio --aid=1 (*) (aac 2ch 48000Hz)
[vo/gpu] opengl cocoa backend is deprecated, use vo=libmpv instead
AO: [coreaudio] 48000Hz stereo 2ch floatp
VO: [gpu] 768x432 yuv420p
[stream.segmented][debug] Closing worker thread
[stream.segmented][debug] Closing worker thread
[stream.segmented][debug] Closing writer thread
[stream.segmented][debug] Closing writer thread
[stream.ffmpegmux][debug] Pipe copy complete: /var/tmp/streamlinkpipe-55925-2-8564
[stream.ffmpegmux][debug] Pipe copy complete: /var/tmp/streamlinkpipe-55925-1-9651
[stream.ffmpegmux][debug] Closing ffmpeg thread
[stream.ffmpegmux][debug] Closed all the substreams
[cli][info] Stream ended
AV: 00:00:30 / 00:00:30 (100%) A-V: -0.411
Invalid video timestamp: 30.163000 -> 29.996000
AV: 00:00:30 / 00:00:30 (100%) A-V: -0.244
Invalid video timestamp: 29.996000 -> 29.996000
AV: 00:00:29 / 00:00:30 (99%) A-V: -0.294 Dropped: 1
Invalid video timestamp: 29.996000 -> 29.996000
AV: 00:00:29 / 00:00:30 (99%) A-V: -0.294 Dropped: 2
*** snipped ***
Invalid video timestamp: 29.996000 -> 29.996000
AV: 00:00:29 / 00:00:30 (99%) A-V: -0.294 Dropped: 892
Invalid video timestamp: 29.996000 -> 29.996000
AV: 00:00:29 / 00:00:30 (99%) A-V: 0.000 ct: -0.104 Dropped: 896
Exiting... (End of file)
[cli][info] Closing currently open stream...
```
| 2023-02-22T23:36:10 |
|
streamlink/streamlink | 5,193 | streamlink__streamlink-5193 | [
"5192"
] | 77e67a7002308dbb41a66c82f6e6fd6a4ff66e2b | diff --git a/src/streamlink/session.py b/src/streamlink/session.py
--- a/src/streamlink/session.py
+++ b/src/streamlink/session.py
@@ -32,16 +32,6 @@ class PythonDeprecatedWarning(UserWarning):
class StreamlinkOptions(Options):
- _OPTIONS_HTTP_ATTRS = {
- "http-cookies": "cookies",
- "http-headers": "headers",
- "http-query-params": "params",
- "http-ssl-cert": "cert",
- "http-ssl-trust-env": "trust_env",
- "http-ssl-verify": "verify",
- "http-timeout": "timeout",
- }
-
def __init__(self, session: "Streamlink", *args, **kwargs):
super().__init__(*args, **kwargs)
self.session = session
@@ -144,6 +134,16 @@ def inner(self: "StreamlinkOptions", key: str, value: Any) -> None:
# ----
+ _OPTIONS_HTTP_ATTRS = {
+ "http-cookies": "cookies",
+ "http-headers": "headers",
+ "http-query-params": "params",
+ "http-ssl-cert": "cert",
+ "http-ssl-verify": "verify",
+ "http-trust-env": "trust_env",
+ "http-timeout": "timeout",
+ }
+
_MAP_GETTERS: ClassVar[Mapping[str, Callable[["StreamlinkOptions", str], Any]]] = {
"http-proxy": _get_http_proxy,
"https-proxy": _get_http_proxy,
| diff --git a/tests/test_session.py b/tests/test_session.py
--- a/tests/test_session.py
+++ b/tests/test_session.py
@@ -525,6 +525,24 @@ def test_string(self, session: Streamlink, option: str, value: str):
session.set_option(option, value)
[email protected](
+ ("option", "attr", "default", "value"),
+ [
+ ("http-ssl-cert", "cert", None, "foo"),
+ ("http-ssl-verify", "verify", True, False),
+ ("http-trust-env", "trust_env", True, False),
+ ("http-timeout", "timeout", 20.0, 30.0),
+ ],
+)
+def test_options_http_other(session: Streamlink, option: str, attr: str, default, value):
+ httpsessionattr = getattr(session.http, attr)
+ assert httpsessionattr == default
+ assert session.get_option(option) == httpsessionattr
+
+ session.set_option(option, value)
+ assert session.get_option(option) == value
+
+
class TestOptionsDocumentation:
@pytest.fixture()
def docstring(self, session: Streamlink):
| Use of CLI parameter --http-ignore-env causes KeyError on all streams
### Checklist
- [X] This is a bug report and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed bug reports](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22bug%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest build from the master branch
### Description
When using the parameter **--http-ignore-env** to ignore local environment variables for proxy, this always errors on any stream in the current/latest release. This worked on previous releases with no issues.
Any use of this CLI option gives an error `KeyError: 'http-trust-env'`
Test results below are from a common open non-DRM stream for m3u8 testing of
https://demo.unified-streaming.com/k8s/features/stable/video/tears-of-steel/tears-of-steel.ism/.m3u8
Test results were performed on Debian Bookworm with Python 3.11.2 and Streamlink 5.3.0+11.g77e67a70
### Debug log
```text
When using the optional CLI parameter of "--http-ignore-env" this errors as per the command output below
streamlink --loglevel=debug --http-ignore-env https://demo.unified-streaming.com/k8s/features/stable/video/tears-of-steel/tears-of-steel.ism/.m3u8 350p
[cli][debug] OS: Linux-6.1.0-3-amd64-x86_64-with-glibc2.36
[cli][debug] Python: 3.11.2
[cli][debug] Streamlink: 5.3.0+11.g77e67a70
[cli][debug] Dependencies:
[cli][debug] certifi: 2022.12.7
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.2
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.17
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.28.2
[cli][debug] urllib3: 1.26.14
[cli][debug] websocket-client: 1.5.1
[cli][debug] Arguments:
[cli][debug] url=https://demo.unified-streaming.com/k8s/features/stable/video/tears-of-steel/tears-of-steel.ism/.m3u8
[cli][debug] stream=['350p']
[cli][debug] --loglevel=debug
[cli][debug] --http-ignore-env=True
Traceback (most recent call last):
File "/home/mason/.myenv/streamlink/bin/streamlink", line 8, in <module>
sys.exit(main())
^^^^^^
File "/home/mason/.myenv/streamlink/lib/python3.11/site-packages/streamlink_cli/main.py", line 895, in main
setup_session_options(streamlink, args)
File "/home/mason/.myenv/streamlink/lib/python3.11/site-packages/streamlink_cli/argparser.py", line 1294, in setup_session_options
session.set_option(option, value)
File "/home/mason/.myenv/streamlink/lib/python3.11/site-packages/streamlink/session.py", line 473, in set_option
self.options.set(key, value)
File "/home/mason/.myenv/streamlink/lib/python3.11/site-packages/streamlink/options.py", line 54, in set
method(self, normalized, value)
File "/home/mason/.myenv/streamlink/lib/python3.11/site-packages/streamlink/session.py", line 108, in _set_http_attr
setattr(self.session.http, self._OPTIONS_HTTP_ATTRS[key], value)
~~~~~~~~~~~~~~~~~~~~~~~~^^^^^
KeyError: 'http-trust-env'
Exact same command without that CLI option works fine as per below.
streamlink --loglevel=debug https://demo.unified-streaming.com/k8s/features/stable/video/tears-of-steel/tears-of-steel.ism/.m3u8 350p
[cli][debug] OS: Linux-6.1.0-3-amd64-x86_64-with-glibc2.36
[cli][debug] Python: 3.11.2
[cli][debug] Streamlink: 5.3.0+11.g77e67a70
[cli][debug] Dependencies:
[cli][debug] certifi: 2022.12.7
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.2
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.17
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.28.2
[cli][debug] urllib3: 1.26.14
[cli][debug] websocket-client: 1.5.1
[cli][debug] Arguments:
[cli][debug] url=https://demo.unified-streaming.com/k8s/features/stable/video/tears-of-steel/tears-of-steel.ism/.m3u8
[cli][debug] stream=['350p']
[cli][debug] --loglevel=debug
[cli][info] Found matching plugin hls for URL https://demo.unified-streaming.com/k8s/features/stable/video/tears-of-steel/tears-of-steel.ism/.m3u8
[plugins.hls][debug] URL=https://demo.unified-streaming.com/k8s/features/stable/video/tears-of-steel/tears-of-steel.ism/.m3u8; params={}
[utils.l10n][debug] Language code: en_GB
[cli][info] Available streams: 68k (worst), 140k, 100p, 200p, 350p, 750p_alt, 750p (best)
[cli][info] Opening stream: 350p (hls)
[cli][info] Starting player: /usr/bin/vlc
[stream.hls][debug] Reloading playlist
[cli][debug] Pre-buffering 8192 bytes
[stream.hls][debug] First Sequence: 1; Last Sequence: 184
[stream.hls][debug] Start offset: 0; Duration: None; Start Sequence: 1; End Sequence: 184
[stream.hls][debug] Adding segment 1 to queue
[stream.hls][debug] Adding segment 2 to queue
```
| That has nothing to do with any stream or plugin implementations, it's a bug in the session initialization.
> This worked on previous releases with no issues.
Looks like this was introduced in 5.2.0 via #5076
https://github.com/streamlink/streamlink/blame/77e67a7002308dbb41a66c82f6e6fd6a4ff66e2b/src/streamlink/session.py#L40
The options key needs to be `http-trust-env`, not `http-ssl-trust-env`. | 2023-02-23T21:06:42 |
streamlink/streamlink | 5,194 | streamlink__streamlink-5194 | [
"4586"
] | 2a24f314f2754f4226f446f583b6fb728574cb71 | diff --git a/src/streamlink/stream/dash_manifest.py b/src/streamlink/stream/dash_manifest.py
--- a/src/streamlink/stream/dash_manifest.py
+++ b/src/streamlink/stream/dash_manifest.py
@@ -613,9 +613,9 @@ def __init__(self, node, root=None, parent=None, *args, **kwargs):
# children
self.segmentTimeline = self.only_child(SegmentTimeline)
- def segments(self, **kwargs) -> Iterator[Segment]:
+ def segments(self, base_url: str, **kwargs) -> Iterator[Segment]:
if kwargs.pop("init", True):
- init_url = self.format_initialization(**kwargs)
+ init_url = self.format_initialization(base_url, **kwargs)
if init_url:
yield Segment(
url=init_url,
@@ -623,7 +623,7 @@ def segments(self, **kwargs) -> Iterator[Segment]:
init=True,
content=False,
)
- for media_url, available_at in self.format_media(**kwargs):
+ for media_url, available_at in self.format_media(base_url, **kwargs):
yield Segment(
url=media_url,
duration=self.duration_seconds,
@@ -632,18 +632,13 @@ def segments(self, **kwargs) -> Iterator[Segment]:
available_at=available_at,
)
- def make_url(self, url: str) -> str:
- """
- Join the URL with the base URL, unless it's an absolute URL
- :param url: maybe relative URL
- :return: joined URL
- """
-
- return BaseURL.join(self.base_url, url)
+ @staticmethod
+ def make_url(base_url: str, url: str) -> str:
+ return BaseURL.join(base_url, url)
- def format_initialization(self, **kwargs) -> Optional[str]:
+ def format_initialization(self, base_url: str, **kwargs) -> Optional[str]:
if self.initialization:
- return self.make_url(self.initialization(**kwargs))
+ return self.make_url(base_url, self.initialization(**kwargs))
def segment_numbers(self) -> Iterator[Tuple[int, datetime.datetime]]:
"""
@@ -698,10 +693,10 @@ def segment_numbers(self) -> Iterator[Tuple[int, datetime.datetime]]:
yield from zip(number_iter, available_iter)
- def format_media(self, **kwargs) -> Iterator[Tuple[str, datetime.datetime]]:
+ def format_media(self, base_url: str, **kwargs) -> Iterator[Tuple[str, datetime.datetime]]:
if not self.segmentTimeline:
for number, available_at in self.segment_numbers():
- url = self.make_url(self.media(Number=number, **kwargs))
+ url = self.make_url(base_url, self.media(Number=number, **kwargs))
yield url, available_at
return
@@ -714,7 +709,7 @@ def format_media(self, **kwargs) -> Iterator[Tuple[str, datetime.datetime]]:
if self.root.type == "static":
for segment, n in zip(self.segmentTimeline.segments, count(self.startNumber)):
- url = self.make_url(self.media(Time=segment.t, Number=n, **kwargs))
+ url = self.make_url(base_url, self.media(Time=segment.t, Number=n, **kwargs))
available_at = datetime.datetime.now(tz=UTC) # TODO: replace with EPOCH_START ?!
yield url, available_at
return
@@ -731,7 +726,7 @@ def format_media(self, **kwargs) -> Iterator[Tuple[str, datetime.datetime]]:
# the last segment in the timeline is the most recent one
# so, work backwards and calculate when each of the segments was
# available, based on the durations relative to the publish-time
- url = self.make_url(self.media(Time=segment.t, Number=n, **kwargs))
+ url = self.make_url(base_url, self.media(Time=segment.t, Number=n, **kwargs))
duration = datetime.timedelta(seconds=segment.d / self.timescale)
# once the suggested_delay is reach stop
@@ -830,6 +825,7 @@ def segments(self, **kwargs) -> Iterator[Segment]:
if segmentTemplate:
yield from segmentTemplate.segments(
+ self.base_url,
RepresentationID=self.id,
Bandwidth=int(self.bandwidth * 1000),
**kwargs,
| diff --git a/tests/resources/dash/test_nested_baseurls.mpd b/tests/resources/dash/test_nested_baseurls.mpd
new file mode 100644
--- /dev/null
+++ b/tests/resources/dash/test_nested_baseurls.mpd
@@ -0,0 +1,67 @@
+<?xml version="1.0" encoding="UTF-8"?>
+<MPD
+ xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
+ xmlns:xlink="http://www.w3.org/1999/xlink"
+ xmlns="urn:mpeg:dash:schema:mpd:2011"
+ xsi:schemaLocation="urn:mpeg:DASH:schema:MPD:2011 http://standards.iso.org/ittf/PubliclyAvailableStandards/MPEG-DASH_schema_files/DASH-MPD.xsd"
+ profiles="urn:mpeg:dash:profile:isoff-live:2011"
+ type="static"
+ mediaPresentationDuration="PT0H0M6.00S"
+ minBufferTime="PT6.0S"
+>
+ <BaseURL>https://hostname/</BaseURL>
+ <Period id="0" start="PT0.0S">
+ <BaseURL>period/</BaseURL>
+ <AdaptationSet
+ id="0"
+ mimeType="video/mp4"
+ maxWidth="1920"
+ maxHeight="1080"
+ par="16:9"
+ frameRate="60"
+ segmentAlignment="true"
+ startWithSAP="1"
+ subsegmentAlignment="true"
+ subsegmentStartsWithSAP="1"
+ >
+ <SegmentTemplate
+ presentationTimeOffset="0"
+ timescale="90000"
+ duration="540000"
+ startNumber="1"
+ media="media_$RepresentationID$-$Number$.m4s"
+ initialization="init_$RepresentationID$.m4s"
+ />
+ <Representation id="video_5000kbps" codecs="avc1.640028" width="1920" height="1080" sar="1:1" bandwidth="5000000"/>
+ <Representation id="video_9000kbps" codecs="avc1.640028" width="1920" height="1080" sar="1:1" bandwidth="9000000">
+ <BaseURL>representation/</BaseURL>
+ </Representation>
+ </AdaptationSet>
+ <AdaptationSet
+ id="1"
+ mimeType="audio/mp4"
+ lang="eng"
+ segmentAlignment="true"
+ startWithSAP="1"
+ subsegmentAlignment="true"
+ subsegmentStartsWithSAP="1"
+ >
+ <BaseURL>adaptationset/</BaseURL>
+ <SegmentTemplate
+ presentationTimeOffset="0"
+ timescale="44100"
+ duration="264600"
+ startNumber="1"
+ media="media_$RepresentationID$-$Number$.m4s"
+ initialization="init_$RepresentationID$.m4s"
+ />
+ <Representation id="audio_128kbps" codecs="mp4a.40.2" audioSamplingRate="44100" sar="1:1" bandwidth="128000"/>
+ <Representation id="audio_256kbps" codecs="mp4a.40.2" audioSamplingRate="44100" sar="1:1" bandwidth="256000">
+ <BaseURL>representation/</BaseURL>
+ </Representation>
+ <Representation id="audio_320kbps" codecs="mp4a.40.2" audioSamplingRate="44100" sar="1:1" bandwidth="320000">
+ <BaseURL>https://other/</BaseURL>
+ </Representation>
+ </AdaptationSet>
+ </Period>
+</MPD>
diff --git a/tests/stream/test_dash_parser.py b/tests/stream/test_dash_parser.py
--- a/tests/stream/test_dash_parser.py
+++ b/tests/stream/test_dash_parser.py
@@ -287,3 +287,33 @@ def test_segments_byterange(self):
("http://test/audio-frag.mp4", False, (374366, 471)),
],
]
+
+ def test_nested_baseurls(self):
+ with xml("dash/test_nested_baseurls.mpd") as mpd_xml:
+ mpd = MPD(mpd_xml, base_url="https://foo/", url="https://test/manifest.mpd")
+ segment_urls = [
+ [seg.url for seg in itertools.islice(representation.segments(), 2)]
+ for adaptationset in mpd.periods[0].adaptationSets for representation in adaptationset.representations
+ ]
+ assert segment_urls == [
+ [
+ "https://hostname/period/init_video_5000kbps.m4s",
+ "https://hostname/period/media_video_5000kbps-1.m4s",
+ ],
+ [
+ "https://hostname/period/representation/init_video_9000kbps.m4s",
+ "https://hostname/period/representation/media_video_9000kbps-1.m4s",
+ ],
+ [
+ "https://hostname/period/adaptationset/init_audio_128kbps.m4s",
+ "https://hostname/period/adaptationset/media_audio_128kbps-1.m4s",
+ ],
+ [
+ "https://hostname/period/adaptationset/representation/init_audio_256kbps.m4s",
+ "https://hostname/period/adaptationset/representation/media_audio_256kbps-1.m4s",
+ ],
+ [
+ "https://other/init_audio_320kbps.m4s",
+ "https://other/media_audio_320kbps-1.m4s",
+ ],
+ ]
| stream.dash: nested BaseURL resolving
### Checklist
- [X] This is a plugin issue and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest build from the master branch
### Description
This specific URL works with mpv, totem and ffplay, but doesn't with vlc and streamlink:
``https://rfe-ingest.akamaized.net/dash/live/2033030/tvmc05/manifest.mpd``
### Debug log
```text
[cli][debug] OS: Linux-5.10.0-15-amd64-x86_64-with-glibc2.31
[cli][debug] Python: 3.9.2
[cli][debug] Streamlink: 4.1.0+12.g0dd52932
[cli][debug] Dependencies:
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.8.0
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.14.1
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.27.1
[cli][debug] websocket-client: 1.3.2
[cli][debug] Arguments:
[cli][debug] url=https://rfe-ingest.akamaized.net/dash/live/2033030/tvmc05/manifest.mpd
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --player=totem
[cli][info] Found matching plugin dash for URL https://rfe-ingest.akamaized.net/dash/live/2033030/tvmc05/manifest.mpd
[plugins.dash][debug] URL=https://rfe-ingest.akamaized.net/dash/live/2033030/tvmc05/manifest.mpd; params={}
[utils.l10n][debug] Language code: en_US
[stream.dash][debug] Available languages for DASH audio streams: eng (using: eng)
[cli][info] Available streams: 240p (worst), 360p, 480p, 720p, 1080p (best)
[cli][info] Opening stream: 1080p (dash)
[cli][info] Starting player: totem
[stream.dash][debug] Opening DASH reader for: video_3311kbps (video/mp4)
[stream.dash][debug] Opening DASH reader for: audio_1 (audio/mp4)
[stream.dash_manifest][debug] Generating segment numbers for dynamic playlist (id=0)
[cli][error] Try 1/1: Could not open stream <DASHStream ['dash', 'https://rfe-ingest.akamaized.net/dash/live/2033030/tvmc05/manifest.mpd']> (Could not open stream: cannot use FFMPEG)
[stream.ffmpegmux][debug] Closing ffmpeg thread
error: Could not open stream <DASHStream ['dash', 'https://rfe-ingest.akamaized.net/dash/live/2033030/tvmc05/manifest.mpd']>, tried 1 times, exiting
[stream.dash_manifest][debug] Generating segment numbers for dynamic playlist (id=1)
Exception in thread Thread-DASHStreamWorker:
Traceback (most recent call last):
File "/usr/lib/python3.9/threading.py", line 954, in _bootstrap_inner
self.run()
File "/usr/local/lib/python3.9/dist-packages/streamlink/stream/segmented.py", line 87, in run
self.writer.put(segment)
File "/usr/local/lib/python3.9/dist-packages/streamlink/stream/segmented.py", line 143, in put
future = self.executor.submit(self.fetch, segment, retries=self.retries)
File "/usr/lib/python3.9/concurrent/futures/thread.py", line 163, in submit
raise RuntimeError('cannot schedule new futures after '
RuntimeError: cannot schedule new futures after interpreter shutdown
[stream.dash][error] Failed to open segment https://rfe-ingest.akamaized.net/dash/live/2033030/tvmc05/init_video_3311kbps.m4s: Unable to open URL: https://rfe-ingest.akamaized.net/dash/live/2033030/tvmc05/init_video_3311kbps.m4s (404 Client Error: Not Found for url: https://rfe-ingest.akamaized.net/dash/live/2033030/tvmc05/init_video_3311kbps.m4s)
```
| > `https://rfe-ingest.akamaized.net/dash/live/2033030/tvmc05/init_video_3311kbps.m4s`
Looks like the `Representation`'s `BaseURL` gets ignored. The URL of the initialization should be
`https://rfe-ingest.akamaized.net/dash/live/2033030/tvmc05/RFE-TVMC05_0240/12wxxnb2/init_video_238kbps.m4s`
```xml
...
<BaseURL>https://rfe-ingest.akamaized.net/dash/live/2033030/tvmc05/</BaseURL>
<Period id="0" start="PT0.0S">
<AdaptationSet id="0" mimeType="video/mp4" maxWidth="1920" maxHeight="1080" par="16:9" frameRate="25" segmentAlignment="true" startWithSAP="1" subsegmentAlignment="true" subsegmentStartsWithSAP="1">
<SegmentTemplate presentationTimeOffset="0" timescale="90000" duration="540000" startNumber="1" media="media_$RepresentationID$-$Number$.m4s" initialization="init_$RepresentationID$.m4s"/>
<Representation id="video_238kbps" codecs="avc1.42c015" width="426" height="240" sar="1:1" bandwidth="238000">
<BaseURL>RFE-TVMC05_0240/12wxxnb2/</BaseURL>
</Representation>
...
```
The issue is caused by the DASH parser not resolving nested BaseURLs on the
1. document level
2. MPD level
3. Period level
4. AdaptationSet level
5. Representation level
This either needs to be fixed in `MPDNode.base_url` where it currently only takes the current level's `BaseURL` into consideration
https://github.com/streamlink/streamlink/blob/07bd742ddff22dd95ef2c90a64bedfdb0a1bd082/src/streamlink/stream/dash_manifest.py#L177-L182
or better, in the `BaseURL(MPDNode)` class, where it only has to resolve it once from its parent/ancestor nodes
https://github.com/streamlink/streamlink/blob/07bd742ddff22dd95ef2c90a64bedfdb0a1bd082/src/streamlink/stream/dash_manifest.py#L270-L293 | 2023-02-24T00:10:33 |
streamlink/streamlink | 5,206 | streamlink__streamlink-5206 | [
"5202"
] | 34c5f5ee5953412c6214ad4a3c18dd08d1229c24 | diff --git a/src/streamlink/plugins/tv5monde.py b/src/streamlink/plugins/tv5monde.py
--- a/src/streamlink/plugins/tv5monde.py
+++ b/src/streamlink/plugins/tv5monde.py
@@ -3,80 +3,93 @@
$url tv5monde.com
$url tivi5mondeplus.com
$type live, vod
-$region France, Belgium, Switzerland
+$region France, Belgium, Switzerland, Africa
"""
import re
-from urllib.parse import urlparse
+from urllib.parse import quote
-from streamlink.plugin import Plugin, PluginError, pluginmatcher
+from streamlink.plugin import Plugin, pluginmatcher
from streamlink.plugin.api import validate
+from streamlink.stream.dash import DASHStream
from streamlink.stream.hls import HLSStream
from streamlink.stream.http import HTTPStream
from streamlink.utils.url import update_scheme
-@pluginmatcher(re.compile(r"""
- https?://(?:[\w-]+\.)*(?:tv5monde|tivi5mondeplus)\.com/
-""", re.VERBOSE))
+@pluginmatcher(re.compile(
+ r"https?://(?:[\w-]+\.)*(?:tv5monde|tivi5mondeplus)\.com/",
+))
class TV5Monde(Plugin):
- def _get_hls(self, root):
- schema_live = validate.Schema(
- validate.xml_xpath_string(".//*[contains(@data-broadcast,'m3u8')]/@data-broadcast"),
- str,
- validate.parse_json(),
- validate.any(
- validate.all({"files": list}, validate.get("files")),
- list,
+ _URL_API = "https://api.tv5monde.com/player/asset/{asset}/resolve"
+
+ _MAP_STREAMTYPES = {
+ "application/x-mpegURL": HLSStream.parse_variant_playlist,
+ "application/dash+xml": DASHStream.parse_manifest,
+ "video/mp4": lambda *args: {"vod": HTTPStream(*args)},
+ }
+
+ def _find_streams(self, data):
+ schema_streams = validate.Schema([
+ validate.all(
+ {
+ "type": str,
+ "url": validate.url(),
+ },
+ validate.union_get("type", "url"),
),
- [{
- "url": validate.url(path=validate.endswith(".m3u8")),
- }],
- validate.get((0, "url")),
- validate.transform(lambda content_url: update_scheme("https://", content_url)),
+ ])
+ streams = schema_streams.validate(data)
+
+ for mimetype, streamfactory in self._MAP_STREAMTYPES.items():
+ for streamtype, streamurl in streams:
+ if streamtype == mimetype:
+ url = update_scheme("https://", streamurl)
+ return streamfactory(self.session, url)
+
+ def _get_playlist(self, data):
+ schema_playlist = validate.Schema(
+ validate.parse_json(),
+ [{"sources": list}],
+ validate.get((0, "sources")),
)
- try:
- live = schema_live.validate(root)
- except PluginError:
- return
-
- return HLSStream.parse_variant_playlist(self.session, live)
-
- def _get_vod(self, root):
- schema_vod = validate.Schema(
- validate.xml_xpath_string(".//script[@type='application/ld+json'][contains(text(),'VideoObject')][1]/text()"),
- str,
- validate.transform(lambda jsonlike: re.sub(r"[\r\n]+", "", jsonlike)),
+ playlist = schema_playlist.validate(data)
+
+ return self._find_streams(playlist)
+
+ def _get_broadcast(self, data):
+ schema_broadcast = validate.Schema(
validate.parse_json(),
- validate.any(
- validate.all(
- {"@graph": [dict]},
- validate.get("@graph"),
- validate.filter(lambda obj: obj["@type"] == "VideoObject"),
- validate.get(0),
- ),
- dict,
- ),
- {"contentUrl": validate.url()},
- validate.get("contentUrl"),
- validate.transform(lambda content_url: update_scheme("https://", content_url)),
+ [{
+ "type": str,
+ "url": str,
+ }],
)
- try:
- vod = schema_vod.validate(root)
- except PluginError:
- return
+ broadcast = schema_broadcast.validate(data)
- if urlparse(vod).path.endswith(".m3u8"):
- return HLSStream.parse_variant_playlist(self.session, vod)
+ deferred = next((item["url"] for item in broadcast if item["type"] == "application/deferred"), None)
+ if deferred:
+ url = self._URL_API.format(asset=quote(deferred))
+ broadcast = self.session.http.get(url, schema=validate.Schema(
+ validate.parse_json(),
+ ))
- return {"vod": HTTPStream(self.session, vod)}
+ return self._find_streams(broadcast)
def _get_streams(self):
- root = self.session.http.get(self.url, schema=validate.Schema(
+ playlist, broadcast = self.session.http.get(self.url, schema=validate.Schema(
validate.parse_html(),
+ validate.union((
+ validate.xml_xpath_string(".//*[@data-playlist][1]/@data-playlist"),
+ validate.xml_xpath_string(".//*[@data-broadcast][1]/@data-broadcast"),
+ )),
))
- return self._get_hls(root) or self._get_vod(root)
+ # data-playlist needs higher priority (data-broadcast attribute exists on the same DOM node)
+ if playlist:
+ return self._get_playlist(playlist)
+ if broadcast:
+ return self._get_broadcast(broadcast)
__plugin__ = TV5Monde
| diff --git a/tests/plugins/test_tv5monde.py b/tests/plugins/test_tv5monde.py
--- a/tests/plugins/test_tv5monde.py
+++ b/tests/plugins/test_tv5monde.py
@@ -6,11 +6,11 @@ class TestPluginCanHandleUrlTV5Monde(PluginCanHandleUrl):
__plugin__ = TV5Monde
should_match = [
- "http://live.tv5monde.com/fbs.html",
- "https://www.tv5monde.com/emissions/episode/version-francaise-vf-83",
- "https://revoir.tv5monde.com/toutes-les-videos/cinema/je-ne-reve-que-de-vous",
- "https://revoir.tv5monde.com/toutes-les-videos/documentaires/des-russes-blancs-des-russes-blancs",
+ "https://live.tv5monde.com/fbs.html",
+ "https://europe.tv5monde.com/fr/direct",
+ "https://maghreb-orient.tv5monde.com/fr/direct",
+ "https://revoir.tv5monde.com/toutes-les-videos/documentaires/sauver-notre-dame-sauver-notre-dame-14-04-2020",
"https://information.tv5monde.com/video/la-diplomatie-francaise-est-elle-en-crise",
- "https://afrique.tv5monde.com/videos/exclusivites-web/les-tutos-de-magloire/season-1/episode-1",
+ "https://afrique.tv5monde.com/videos/afrykas-et-la-boite-magique",
"https://www.tivi5mondeplus.com/conte-nous/episode-25",
]
| plugins.tv5monde: broken
### Checklist
- [X] This is a plugin issue and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest stable release
### Description
The TV5Monde is completely outdated and useless with the actuel website and urls. I have to remove it completely after every streamlink update, otherwise i'm not able to use any of the TV5 hls links from the website. So I think it's better to remove it or update it if possible. Thanks.
### Debug log
```text
N/A
```
| > i'm not able to use any of the TV5 hls links from the website
https://streamlink.github.io/cli/protocols.html#playing-built-in-streaming-protocols-directly
```
streamlink 'hls://https://...' best
```
Post a full debug log please, as requested by the issue form
> > i'm not able to use any of the TV5 hls links from the website
>
> https://streamlink.github.io/cli/protocols.html#playing-built-in-streaming-protocols-directly
>
> ```
> streamlink 'hls://https://...' best
> ```
"**otherwise** i'm not able to use any of the TV5 hls links from the website" = which means that with the plugin installed in Streamlink, it will start instantly when trying to watch any TV5 channels and stop any attempt to watch the direct HLS links from the TV5 website. This is why it must be removed OR updated in the next Streamlink update in order to avoid this problem...
No, it does not if you use the `hls://` prefix for any HLS playlist input URLs. The **HLS plugin** [does have two matchers](https://github.com/streamlink/streamlink/blob/5.3.1/src/streamlink/plugins/hls.py#L13-L18), one with the `hls://` prefix and normal priority (which is obviously not used by any other plugin), and one with `.m3u8` URL path suffixes and the low priority. If the **tv5monde plugin** matches first because you're not using the special `hls://` prefix, then this won't work of course.
If the **tv5monde plugin** is broken and can't resolve the right HLS URLs, then it needs fixing. It should only get removed if it can't be fixed or if it violates the plugin requirement rules. As said, post the full debug log when trying to use the tv5monde plugin.
Here is the log, only TV5Monde Europe is not working and need to be updated in the plugin (https://europe.tv5monde.com/fr/direct - hls direct link: https://ott.tv5monde.com/Content/HLS/Live/channel(europe)/index.m3u8). The rest is working fine.
TV5Monde France Belgique Suisse Monaco
[cli][info] Found matching plugin tv5monde for URL https://live.tv5monde.com/fbs.html?
[cli][info] Available streams: 216p (worst), 270p, 360p, 540p, 720p, 1080p (best)
[cli][info] Starting server, access with one of:
[cli][info] http://10.10.20.15:129/
[cli][info] http://127.0.0.1:129/
[cli][info] Got HTTP request from VLC/3.0.18 LibVLC/3.0.18
[cli][info] Opening stream: 1080p (hls-multi)
[utils.named_pipe][info] Creating pipe streamlinkpipe-14200-1-6511
[utils.named_pipe][info] Creating pipe streamlinkpipe-14200-2-2045
TV5Monde Europe
[cli][info] Found matching plugin tv5monde for URL https://europe.tv5monde.com/fr/direct
error: No playable streams found on this URL: https://europe.tv5monde.com/fr/direct
TV5Monde Maghreb-Orient
[cli][info] Found matching plugin tv5monde for URL https://maghreb-orient.tv5monde.com/fr/direct
[cli][info] Available streams: 216p (worst), 270p, 360p, 540p, 720p, 1080p (best)
[cli][info] Starting server, access with one of:
[cli][info] http://10.10.20.15:129/
[cli][info] http://10.34.93.116:129/
[cli][info] http://127.0.0.1:129/
[cli][info] Got HTTP request from VLC/3.0.18 LibVLC/3.0.18
[cli][info] Opening stream: 1080p (hls-multi)
[utils.named_pipe][info] Creating pipe streamlinkpipe-14384-1-8345
[utils.named_pipe][info] Creating pipe streamlinkpipe-14384-2-4766
TV5Monde Info
[cli][info] Found matching plugin tv5monde for URL https://maghreb-orient.tv5monde.com/fr/direct-tv5monde-info
[cli][info] Available streams: 216p (worst), 270p, 360p, 540p, 720p, 1080p (best)
[cli][info] Starting server, access with one of:
[cli][info] http://10.10.20.15:129/
[cli][info] http://10.34.93.116:129/
[cli][info] http://127.0.0.1:129/
[cli][info] Got HTTP request from VLC/3.0.18 LibVLC/3.0.18
[cli][info] Opening stream: 1080p (hls-multi)
[utils.named_pipe][info] Creating pipe streamlinkpipe-3372-1-6004
[utils.named_pipe][info] Creating pipe streamlinkpipe-3372-2-7378
Tivi5
[cli][info] Found matching plugin tv5monde for URL https://maghreb-orient.tv5monde.com/fr/direct-tv5-jeunesse
[cli][info] Available streams: 216p (worst), 270p, 360p, 540p, 720p, 1080p (best)
[cli][info] Starting server, access with one of:
[cli][info] http://10.10.20.15:129/
[cli][info] http://10.34.93.116:129/
[cli][info] http://127.0.0.1:129/
[cli][info] Got HTTP request from VLC/3.0.18 LibVLC/3.0.18
[cli][info] Opening stream: 1080p (hls-multi)
[utils.named_pipe][info] Creating pipe streamlinkpipe-8452-1-2605
[utils.named_pipe][info] Creating pipe streamlinkpipe-8452-2-4004
TV5Monde Style
[cli][info] Found matching plugin tv5monde for URL https://maghreb-orient.tv5monde.com/fr/direct-style-hd
[cli][info] Available streams: 216p (worst), 270p, 360p, 540p, 720p, 1080p (best)
[cli][info] Starting server, access with one of:
[cli][info] http://10.10.20.15:129/
[cli][info] http://10.34.93.116:129/
[cli][info] http://127.0.0.1:129/
[cli][info] Got HTTP request from VLC/3.0.18 LibVLC/3.0.18
[cli][info] Opening stream: 1080p (hls-multi)
[utils.named_pipe][info] Creating pipe streamlinkpipe-9144-1-4944
[utils.named_pipe][info] Creating pipe streamlinkpipe-9144-2-4297 | 2023-02-27T00:10:37 |
streamlink/streamlink | 5,217 | streamlink__streamlink-5217 | [
"4607",
"4607"
] | 350cb58a46bad34ce0aa5918572c19061b5f3b8e | diff --git a/src/streamlink/stream/dash_manifest.py b/src/streamlink/stream/dash_manifest.py
--- a/src/streamlink/stream/dash_manifest.py
+++ b/src/streamlink/stream/dash_manifest.py
@@ -622,12 +622,10 @@ def __init__(self, node, root=None, parent=None, period=None, *args, **kwargs):
self.presentationTimeOffset = self.attr(
"presentationTimeOffset",
parser=MPDParsers.timedelta(self.timescale),
+ default=datetime.timedelta(),
)
- if self.duration:
- self.duration_seconds = self.duration / float(self.timescale)
- else:
- self.duration_seconds = None
+ self.duration_seconds = self.duration / self.timescale if self.duration and self.timescale else None
# children
self.segmentTimeline = self.only_child(SegmentTimeline)
@@ -673,6 +671,9 @@ def segment_numbers(self) -> Iterator[Tuple[int, datetime.datetime]]:
In the simplest case, the segment number is based on the time since the availabilityStartTime.
"""
+ if not self.duration_seconds: # pragma: no cover
+ raise MPDParsingError("Unknown segment durations: missing duration/timescale attributes on SegmentTemplate")
+
number_iter: Union[Iterator[int], Sequence[int]]
available_iter: Iterator[datetime.datetime]
@@ -685,29 +686,40 @@ def segment_numbers(self) -> Iterator[Tuple[int, datetime.datetime]]:
number_iter = count(self.startNumber)
else:
now = datetime.datetime.now(UTC)
- if self.presentationTimeOffset:
- since_start = (now - self.presentationTimeOffset) - self.period.availabilityStartTime
- available_start = self.period.availabilityStartTime + self.presentationTimeOffset + since_start
- else:
- since_start = now - self.period.availabilityStartTime
- available_start = now
+ since_start = now - self.period.availabilityStartTime - self.presentationTimeOffset
suggested_delay = self.root.suggestedPresentationDelay
buffer_time = self.root.minBufferTime
- # the number of the segment that is available at NOW - SUGGESTED_DELAY - BUFFER_TIME
- number_offset = int(
- (since_start - suggested_delay - buffer_time).total_seconds()
- / self.duration_seconds,
- )
+ # Segment number
+ # To reduce unnecessary delay, start with the next/upcoming segment: +1
+ seconds_offset = (since_start - suggested_delay - buffer_time).total_seconds()
+ number_offset = max(0, int(seconds_offset / self.duration_seconds) + 1)
number_iter = count(self.startNumber + number_offset)
- # the time the segment number is available at NOW
+ # Segment availability time
+ # The availability time marks the segment's beginning: -1
+ available_offset = datetime.timedelta(seconds=max(0, number_offset - 1) * self.duration_seconds)
+ available_start = self.period.availabilityStartTime + available_offset
available_iter = count_dt(
available_start,
datetime.timedelta(seconds=self.duration_seconds),
)
+ log.debug(f"Stream start: {self.period.availabilityStartTime}")
+ log.debug(f"Current time: {now}")
+ log.debug(f"Availability: {available_start}")
+ log.debug("; ".join([
+ f"presentationTimeOffset: {self.presentationTimeOffset}",
+ f"suggestedPresentationDelay: {self.root.suggestedPresentationDelay}",
+ f"minBufferTime: {self.root.minBufferTime}",
+ ]))
+ log.debug("; ".join([
+ f"segmentDuration: {self.duration_seconds}",
+ f"segmentStart: {self.startNumber}",
+ f"segmentOffset: {number_offset} ({seconds_offset}s)",
+ ]))
+
yield from zip(number_iter, available_iter)
def format_media(self, ident: TTimelineIdent, base_url: str, **kwargs) -> Iterator[Tuple[str, datetime.datetime]]:
| diff --git a/tests/resources/dash/test_segments_dynamic_number.mpd b/tests/resources/dash/test_segments_dynamic_number.mpd
--- a/tests/resources/dash/test_segments_dynamic_number.mpd
+++ b/tests/resources/dash/test_segments_dynamic_number.mpd
@@ -5,15 +5,15 @@
xmlns="urn:mpeg:dash:schema:mpd:2011"
xsi:schemaLocation="urn:mpeg:dash:schema:mpd:2011 http://standards.iso.org/ittf/PubliclyAvailableStandards/MPEG-DASH_schema_files/DASH-MPD.xsd"
type="dynamic"
- availabilityStartTime="2018-05-04T13:20:07Z"
- publishTime="2018-05-10T09:27:09"
+ availabilityStartTime="2000-01-01T00:00:00Z"
+ publishTime="2000-01-01T00:00:00Z"
minimumUpdatePeriod="PT10S"
minBufferTime="PT2S"
suggestedPresentationDelay="PT40S"
timeShiftBufferDepth="PT24H0M0S"
profiles="urn:mpeg:dash:profile:isoff-live:2011"
>
- <Period id="1" start="PT12M34S">
+ <Period id="1" start="PT1M30S">
<AdaptationSet
mimeType="video/mp4"
frameRate="25/1"
@@ -23,24 +23,24 @@
subsegmentStartsWithSAP="1"
bitstreamSwitching="false"
>
- <SegmentTemplate timescale="90000" duration="450000" startNumber="1"/>
+ <SegmentTemplate timescale="90000" duration="450000" startNumber="100"/>
<Representation id="1" width="1280" height="720" bandwidth="2400000" codecs="avc1.64001f">
- <SegmentTemplate duration="450000" startNumber="1" media="hd-5_$Number%09d$.mp4" initialization="hd-5-init.mp4"/>
+ <SegmentTemplate media="hd-5_$Number%09d$.mp4" initialization="hd-5-init.mp4"/>
</Representation>
<Representation id="2" width="1280" height="720" bandwidth="1250000" codecs="avc1.64001f">
- <SegmentTemplate duration="450000" startNumber="1" media="hd-4_$Number%09d$.mp4" initialization="hd-4-init.mp4"/>
+ <SegmentTemplate media="hd-4_$Number%09d$.mp4" initialization="hd-4-init.mp4"/>
</Representation>
<Representation id="3" width="640" height="360" bandwidth="950000" codecs="avc1.4d401e">
- <SegmentTemplate duration="450000" startNumber="1" media="hd-3_$Number%09d$.mp4" initialization="hd-3-init.mp4"/>
+ <SegmentTemplate media="hd-3_$Number%09d$.mp4" initialization="hd-3-init.mp4"/>
</Representation>
<Representation id="4" width="640" height="360" bandwidth="650000" codecs="avc1.4d401e">
- <SegmentTemplate duration="450000" startNumber="1" media="hd-2_$Number%09d$.mp4" initialization="hd-2-init.mp4"/>
+ <SegmentTemplate media="hd-2_$Number%09d$.mp4" initialization="hd-2-init.mp4"/>
</Representation>
<Representation id="5" width="640" height="360" bandwidth="350000" codecs="avc1.4d401e">
- <SegmentTemplate duration="450000" startNumber="1" media="hd-1_$Number%09d$.mp4" initialization="hd-1-init.mp4"/>
+ <SegmentTemplate media="hd-1_$Number%09d$.mp4" initialization="hd-1-init.mp4"/>
</Representation>
<Representation id="6" width="160" height="90" bandwidth="110000" codecs="avc1.4d400b">
- <SegmentTemplate duration="450000" startNumber="1" media="hd-0_$Number%09d$.mp4" initialization="hd-0-init.mp4"/>
+ <SegmentTemplate media="hd-0_$Number%09d$.mp4" initialization="hd-0-init.mp4"/>
</Representation>
</AdaptationSet>
<AdaptationSet
diff --git a/tests/stream/test_dash_parser.py b/tests/stream/test_dash_parser.py
--- a/tests/stream/test_dash_parser.py
+++ b/tests/stream/test_dash_parser.py
@@ -109,8 +109,9 @@ def test_segments_dynamic_time(self):
]
def test_segments_dynamic_number(self):
+ # access manifest one hour after its availabilityStartTime
with xml("dash/test_segments_dynamic_number.mpd") as mpd_xml, \
- freeze_time("2018-05-22T13:37:00Z"):
+ freeze_time("2000-01-01T01:00:00Z"):
mpd = MPD(mpd_xml, base_url="http://test/", url="http://test/manifest.mpd")
stream_urls = [
(segment.url, segment.available_at)
@@ -118,25 +119,21 @@ def test_segments_dynamic_number(self):
]
assert stream_urls == [
- # The initialization segment gets its availability time from
- # the sum of the manifest's availabilityStartTime value and the period's start value, similar to static manifests
(
"http://test/hd-5-init.mp4",
- datetime.datetime(2018, 5, 4, 13, 32, 41, tzinfo=UTC),
+ datetime.datetime(2000, 1, 1, 0, 1, 30, tzinfo=UTC),
),
- # The segment number also takes the availabilityStartTime and period start sum into consideration,
- # but the availability time depends on the current time and the segment durations
(
- "http://test/hd-5_000311084.mp4",
- datetime.datetime(2018, 5, 22, 13, 37, 0, tzinfo=UTC),
+ "http://test/hd-5_000000794.mp4",
+ datetime.datetime(2000, 1, 1, 0, 59, 15, tzinfo=UTC),
),
(
- "http://test/hd-5_000311085.mp4",
- datetime.datetime(2018, 5, 22, 13, 37, 5, tzinfo=UTC),
+ "http://test/hd-5_000000795.mp4",
+ datetime.datetime(2000, 1, 1, 0, 59, 20, tzinfo=UTC),
),
(
- "http://test/hd-5_000311086.mp4",
- datetime.datetime(2018, 5, 22, 13, 37, 10, tzinfo=UTC),
+ "http://test/hd-5_000000796.mp4",
+ datetime.datetime(2000, 1, 1, 0, 59, 25, tzinfo=UTC),
),
]
| stream.dash: failed to create accurate current segment $number$
### Checklist
- [X] This is a bug report and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed bug reports](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22bug%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest stable release
### Description
I am trying to play a Dash stream from "New York". Said stream has segment caching in place they store all segments on there server for 24 hours in order to run the same stream for different time zones.
### MPD structure
```<?xml version="1.0"?>
<MPD xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="urn:mpeg:dash:schema:mpd:2011" xmlns:cenc="urn:mpeg:cenc:2013" xsi:schemaLocation="urn:mpeg:dash:schema:mpd:2011 http://standards.iso.org/ittf/PubliclyAvailableStandards/MPEG-DASH_schema_files/DASH-MPD.xsd" profiles="urn:mpeg:dash:profile:isoff-live:2011,urn:com:dashif:dash264" maxSegmentDuration="PT2.048000S" minBufferTime="PT2.048000S" publishTime="2022-06-17T20:00:00Z" type="dynamic" minimumUpdatePeriod="PT2.048000S" availabilityStartTime="2022-06-17T20:00:15Z" mediaPresentationDuration="PT1800.000000S" suggestedPresentationDelay="PT4.096000S">
<Period id="1" duration="PT1800.000000S" start="PT0.000000S">
<BaseURL>/158/live/55941/</BaseURL>
<AdaptationSet contentType="audio" mimeType="audio/mp4" segmentAlignment="true" startWithSAP="1" codecs="mp4a.40.2" lang="en">
<SegmentTemplate timescale="1000" duration="2048" startNumber="18926" media="audio/$RepresentationID$/$Number%08d$.m4s" presentationTimeOffset="38760243"/>
<AudioChannelConfiguration schemeIdUri="urn:mpeg:dash:23003:3:audio_channel_configuration:2011" value="2"/>
<Role schemeIdUri="urn:mpeg:dash:role:2011" value="main"/>
<Representation id="192a158" audioSamplingRate="48000" bandwidth="196608" qualityRanking="0"/>
</AdaptationSet>
<AdaptationSet contentType="video" mimeType="video/mp4" segmentAlignment="true" startWithSAP="1">
<Accessibility schemeIdUri="urn:scte:dash:cc:cea-608:2015" value="CC1=eng"/>
<Accessibility schemeIdUri="urn:scte:dash:cc:cea-708:2015" value="1=lang:eng"/>
<SegmentTemplate timescale="1000" duration="2048" startNumber="18926" media="video/$RepresentationID$/$Number%08d$.m4s" presentationTimeOffset="38760243"/>
<Role schemeIdUri="urn:mpeg:dash:role:2011" value="main"/>
<Representation id="1080v158" bandwidth="5632000" codecs="avc1.640028" width="1920" height="1080" frameRate="29970/1000" sar="1:1" qualityRanking="0"/>
</AdaptationSet>
</Period>
</MPD>
```
Things to note about this Dash stream is that it is a dynamic live stream that uses the "Number" segment names template and provide a "startNumber" of the current live segment for the manifest.
When i tried to play this stream in "Streamlink" i noticed that the stream was not correct and did not match up with the live stream from there platform. I then tried to play the stream using the "VLC" media player and it plays the correct stream matching up with the platform stream in fact "VLC" was about 10 seconds ahead of the platform stream.
This lead me to believe that the issue with "Streamlink" when debugging the issue there is not much error information provided because the stream still plays with no issues it just plays from cached segment
and not live. To debug and compare both i enabled http/https loggin on my system and checked what segment urls where being called.
Both "VLC" and "Streamlink" where playing the correct base url and stuff but the difference i noticed was that "VLC" was using a segment number higher than the "startNumber" specified in the manifest as it should but "Streamlink" seemed to start streaming from "0" segment number and then going up by one seemingly completely ignoring the "startNumber" specified in the manifest. At the time of creating this Bug report the manifest specified "startNumber" was set to "22442" but stream link was currently trying to play segment number "26".
### Debug log
```text
Debug log contains no use full information other than stream playing as it does play fine just the wrong segment number.
```
### 18/06/2022 Update
This is a screen shot of the streamlink stream and the default mpd link both playing on vlc at the same time
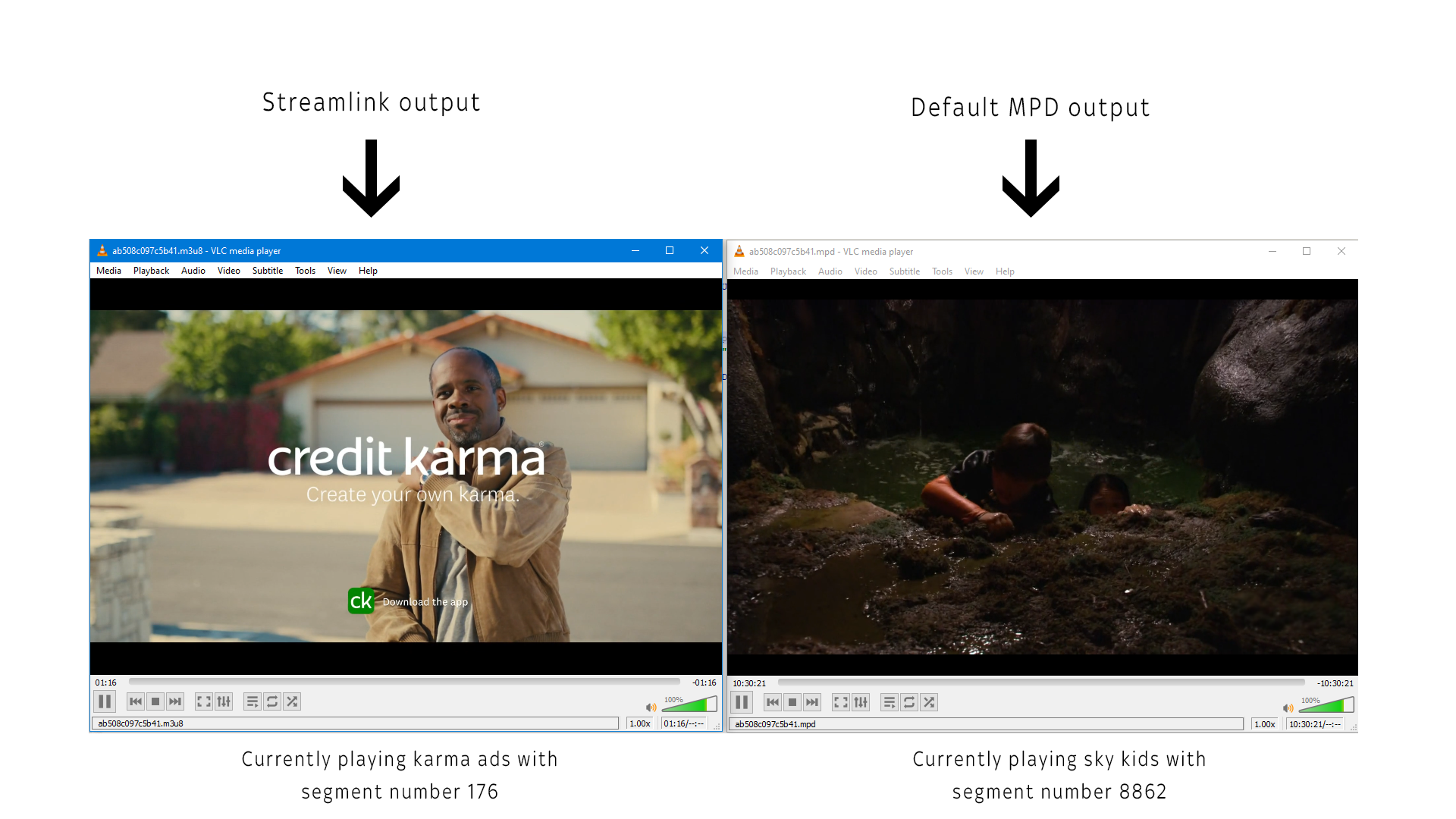
I have done my own calculations in PHP my normal working language and managed to create the correct output from the information provided by the mpd
Sorrty the image below has a small mistake where i forgot to enter the proper manifest delay from the mpd of 4.096 but even with this small error the php code still returns a current segment number a lot closer to the proper number than streamlink is doing on playback.
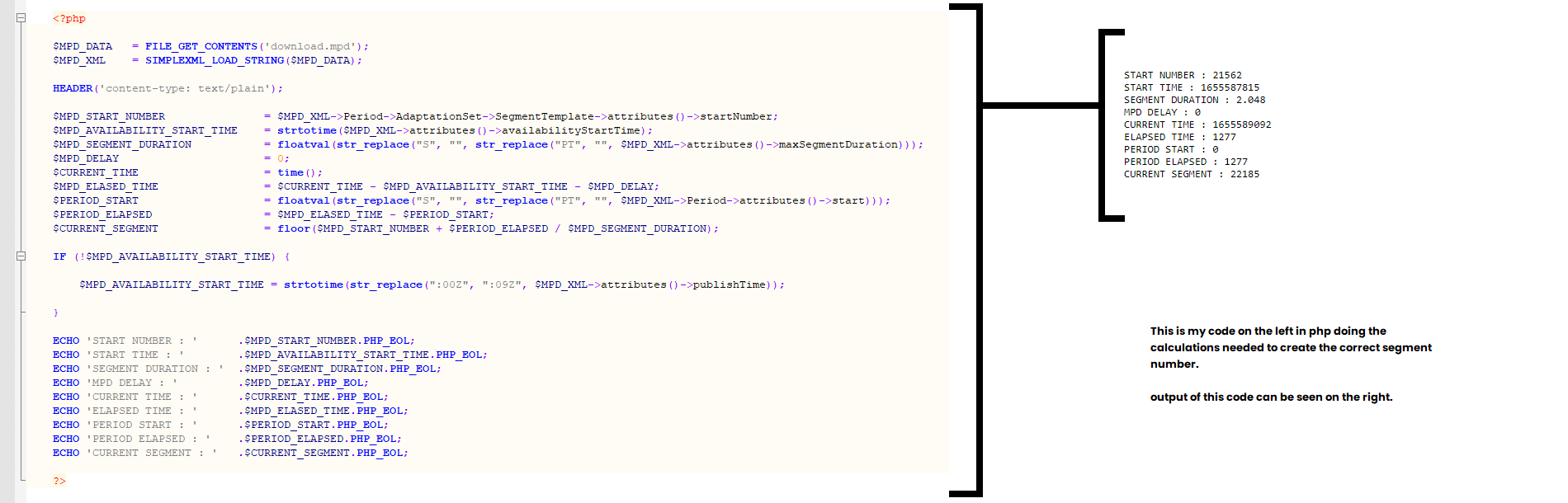
### 19/08/2022 Update
After looking over the code in the MPD manifest as well as the code in "dash_manifest.py" from "streamlink" i can see the error comes from the fact the manifest contains "presentationTimeOffset" where the value is higher than the current segment number so on line 485 of "dash_manifest.py" where it checks for "presentationTimeOffset" it runs the calculations based on this thus we end up with a 0000 value from the calculations because the number is higher than the current segment number value.
I do not know why this number is higher than the current number value i can only guess as to it being used for timezone or playback functions with the custom player platform used by the service.
To get around this i have made some edits to the code in "dash_manifest.py" to check and make sure that it does not lead to a negative/null value when calculating and if it does to skip to the calculations with out the usage of "presentationTimeOffset" and this has now fixed my issue and "streamlink" is now providing the correct numbers for the current playback
To mitigate this issue with possible other services in the future it may be a good option to possibly allow the passing of a arg to ignore the usage of "presentationTimeOffset" when passing a dash source url this way "streamlink" does not need to mess with its current calculations but rather only check for an extra arg and skip said section and use the calculation code with out it.
### End Notes
First of all i would like to that the developers for the amazing software that is "streamlink" it works amazing and allows me to have perfect streaming all over my house on every devices with out needing loads of complicated software and apps.
Second i would like to apologise for any spelling / grammar mistakes in my bug report aka this post i do have dyslexia and i try my best to correct mistakes when i see the red line but it does take for every to go back and forth to google to find the correct spelling so sometimes i skip doing it so am sorry!
stream.dash: failed to create accurate current segment $number$
### Checklist
- [X] This is a bug report and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed bug reports](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22bug%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest stable release
### Description
I am trying to play a Dash stream from "New York". Said stream has segment caching in place they store all segments on there server for 24 hours in order to run the same stream for different time zones.
### MPD structure
```<?xml version="1.0"?>
<MPD xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="urn:mpeg:dash:schema:mpd:2011" xmlns:cenc="urn:mpeg:cenc:2013" xsi:schemaLocation="urn:mpeg:dash:schema:mpd:2011 http://standards.iso.org/ittf/PubliclyAvailableStandards/MPEG-DASH_schema_files/DASH-MPD.xsd" profiles="urn:mpeg:dash:profile:isoff-live:2011,urn:com:dashif:dash264" maxSegmentDuration="PT2.048000S" minBufferTime="PT2.048000S" publishTime="2022-06-17T20:00:00Z" type="dynamic" minimumUpdatePeriod="PT2.048000S" availabilityStartTime="2022-06-17T20:00:15Z" mediaPresentationDuration="PT1800.000000S" suggestedPresentationDelay="PT4.096000S">
<Period id="1" duration="PT1800.000000S" start="PT0.000000S">
<BaseURL>/158/live/55941/</BaseURL>
<AdaptationSet contentType="audio" mimeType="audio/mp4" segmentAlignment="true" startWithSAP="1" codecs="mp4a.40.2" lang="en">
<SegmentTemplate timescale="1000" duration="2048" startNumber="18926" media="audio/$RepresentationID$/$Number%08d$.m4s" presentationTimeOffset="38760243"/>
<AudioChannelConfiguration schemeIdUri="urn:mpeg:dash:23003:3:audio_channel_configuration:2011" value="2"/>
<Role schemeIdUri="urn:mpeg:dash:role:2011" value="main"/>
<Representation id="192a158" audioSamplingRate="48000" bandwidth="196608" qualityRanking="0"/>
</AdaptationSet>
<AdaptationSet contentType="video" mimeType="video/mp4" segmentAlignment="true" startWithSAP="1">
<Accessibility schemeIdUri="urn:scte:dash:cc:cea-608:2015" value="CC1=eng"/>
<Accessibility schemeIdUri="urn:scte:dash:cc:cea-708:2015" value="1=lang:eng"/>
<SegmentTemplate timescale="1000" duration="2048" startNumber="18926" media="video/$RepresentationID$/$Number%08d$.m4s" presentationTimeOffset="38760243"/>
<Role schemeIdUri="urn:mpeg:dash:role:2011" value="main"/>
<Representation id="1080v158" bandwidth="5632000" codecs="avc1.640028" width="1920" height="1080" frameRate="29970/1000" sar="1:1" qualityRanking="0"/>
</AdaptationSet>
</Period>
</MPD>
```
Things to note about this Dash stream is that it is a dynamic live stream that uses the "Number" segment names template and provide a "startNumber" of the current live segment for the manifest.
When i tried to play this stream in "Streamlink" i noticed that the stream was not correct and did not match up with the live stream from there platform. I then tried to play the stream using the "VLC" media player and it plays the correct stream matching up with the platform stream in fact "VLC" was about 10 seconds ahead of the platform stream.
This lead me to believe that the issue with "Streamlink" when debugging the issue there is not much error information provided because the stream still plays with no issues it just plays from cached segment
and not live. To debug and compare both i enabled http/https loggin on my system and checked what segment urls where being called.
Both "VLC" and "Streamlink" where playing the correct base url and stuff but the difference i noticed was that "VLC" was using a segment number higher than the "startNumber" specified in the manifest as it should but "Streamlink" seemed to start streaming from "0" segment number and then going up by one seemingly completely ignoring the "startNumber" specified in the manifest. At the time of creating this Bug report the manifest specified "startNumber" was set to "22442" but stream link was currently trying to play segment number "26".
### Debug log
```text
Debug log contains no use full information other than stream playing as it does play fine just the wrong segment number.
```
### 18/06/2022 Update
This is a screen shot of the streamlink stream and the default mpd link both playing on vlc at the same time
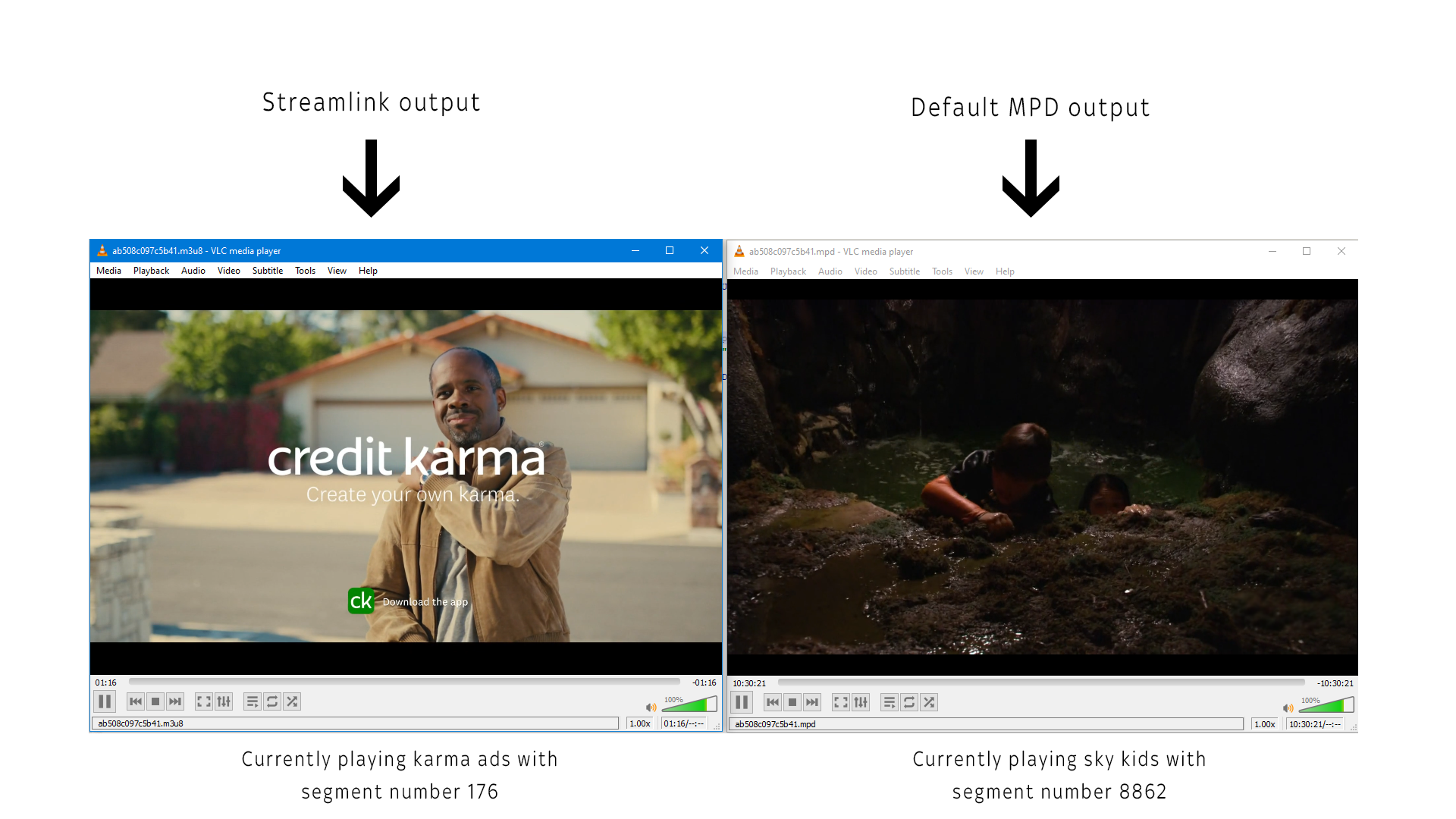
I have done my own calculations in PHP my normal working language and managed to create the correct output from the information provided by the mpd
Sorrty the image below has a small mistake where i forgot to enter the proper manifest delay from the mpd of 4.096 but even with this small error the php code still returns a current segment number a lot closer to the proper number than streamlink is doing on playback.
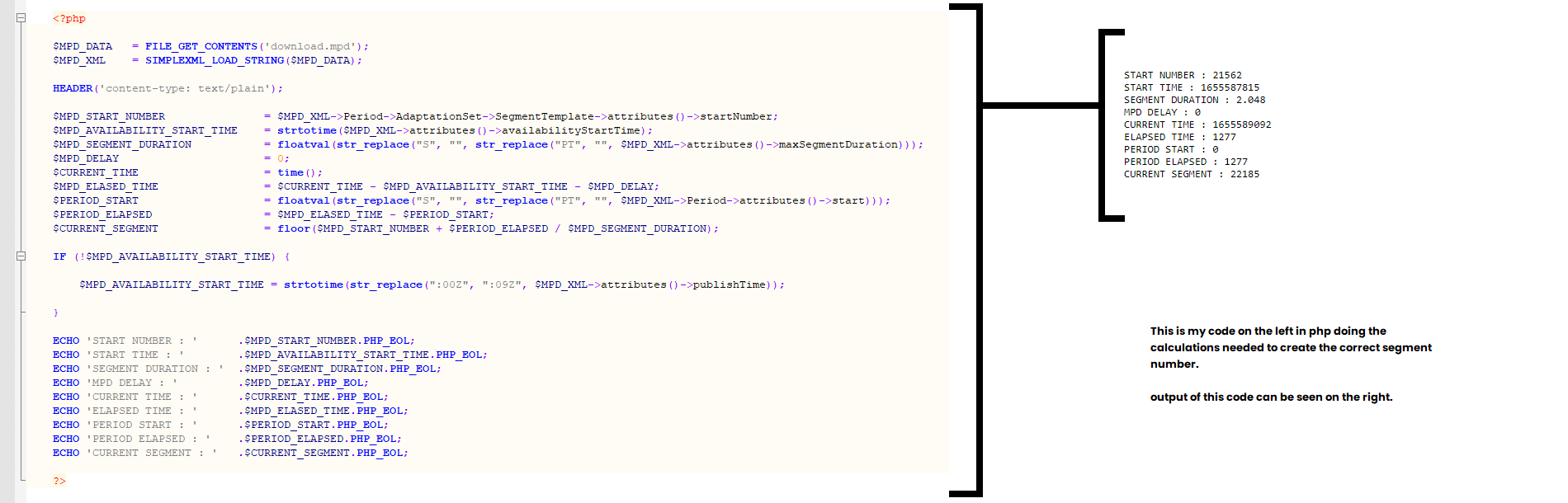
### 19/08/2022 Update
After looking over the code in the MPD manifest as well as the code in "dash_manifest.py" from "streamlink" i can see the error comes from the fact the manifest contains "presentationTimeOffset" where the value is higher than the current segment number so on line 485 of "dash_manifest.py" where it checks for "presentationTimeOffset" it runs the calculations based on this thus we end up with a 0000 value from the calculations because the number is higher than the current segment number value.
I do not know why this number is higher than the current number value i can only guess as to it being used for timezone or playback functions with the custom player platform used by the service.
To get around this i have made some edits to the code in "dash_manifest.py" to check and make sure that it does not lead to a negative/null value when calculating and if it does to skip to the calculations with out the usage of "presentationTimeOffset" and this has now fixed my issue and "streamlink" is now providing the correct numbers for the current playback
To mitigate this issue with possible other services in the future it may be a good option to possibly allow the passing of a arg to ignore the usage of "presentationTimeOffset" when passing a dash source url this way "streamlink" does not need to mess with its current calculations but rather only check for an extra arg and skip said section and use the calculation code with out it.
### End Notes
First of all i would like to that the developers for the amazing software that is "streamlink" it works amazing and allows me to have perfect streaming all over my house on every devices with out needing loads of complicated software and apps.
Second i would like to apologise for any spelling / grammar mistakes in my bug report aka this post i do have dyslexia and i try my best to correct mistakes when i see the red line but it does take for every to go back and forth to google to find the correct spelling so sometimes i skip doing it so am sorry!
| > I did post this in normal discussion as i dont know if its a bug directly as it only seems to happen with these streams only others i have play fine so bit confusing really but it was closed and i was told to post a bug report here.
You were supposed to provide actual information in your bug report. What you have copy&pasted here from your previous post pretty much says "I have tried opening a stream and it didn't work correctly". That isn't something anyone here can debug.
It doesn't matter if you're not sure whether it's a bug. If it's not, then the issue will be closed. If it is, then it can be fixed. But without any useful information, this is just pointless.
Post the URL or stream manifest.
> > I did post this in normal discussion as i dont know if its a bug directly as it only seems to happen with these streams only others i have play fine so bit confusing really but it was closed and i was told to post a bug report here.
>
> You were supposed to provide actual information in your bug report. What you have copy&pasted here from your previous post pretty much says "I have tried opening a stream and it didn't work correctly". That isn't something anyone here can debug.
>
> It doesn't matter if you're not sure whether it's a bug. If it's not, then the issue will be closed. If it is, then it can be fixed. But without any useful information, this is just pointless.
>
> Post the URL or stream manifest.
This is why it was placed into discussion first as i was asking a question aka having a discussion to find out if it was a bug or simply an issue with my selected service!
I was asking how the calcuations for the segment numbers are created so i could compare them to that of vlc and the official provider to see where in lied the issue my self if possible.
As stated there is no debug log because the stream plays perfect it just plays at the wrong time frame and because the stream is rebroadcast for people in different timezones segments are still there and it works so there is no errors to the log.
I will how ever get a copy of the manifest and post it here.
@bastimeyer I have found the cause of the problem and detailed it above i am unsure of how to best go about this situation regarding closing this or marking it as answered with out having someone look over it first in case the team wishes to put a fix or patch in place.
Hopefully i have provided enough detailed information above.
> I did post this in normal discussion as i dont know if its a bug directly as it only seems to happen with these streams only others i have play fine so bit confusing really but it was closed and i was told to post a bug report here.
You were supposed to provide actual information in your bug report. What you have copy&pasted here from your previous post pretty much says "I have tried opening a stream and it didn't work correctly". That isn't something anyone here can debug.
It doesn't matter if you're not sure whether it's a bug. If it's not, then the issue will be closed. If it is, then it can be fixed. But without any useful information, this is just pointless.
Post the URL or stream manifest.
> > I did post this in normal discussion as i dont know if its a bug directly as it only seems to happen with these streams only others i have play fine so bit confusing really but it was closed and i was told to post a bug report here.
>
> You were supposed to provide actual information in your bug report. What you have copy&pasted here from your previous post pretty much says "I have tried opening a stream and it didn't work correctly". That isn't something anyone here can debug.
>
> It doesn't matter if you're not sure whether it's a bug. If it's not, then the issue will be closed. If it is, then it can be fixed. But without any useful information, this is just pointless.
>
> Post the URL or stream manifest.
This is why it was placed into discussion first as i was asking a question aka having a discussion to find out if it was a bug or simply an issue with my selected service!
I was asking how the calcuations for the segment numbers are created so i could compare them to that of vlc and the official provider to see where in lied the issue my self if possible.
As stated there is no debug log because the stream plays perfect it just plays at the wrong time frame and because the stream is rebroadcast for people in different timezones segments are still there and it works so there is no errors to the log.
I will how ever get a copy of the manifest and post it here.
@bastimeyer I have found the cause of the problem and detailed it above i am unsure of how to best go about this situation regarding closing this or marking it as answered with out having someone look over it first in case the team wishes to put a fix or patch in place.
Hopefully i have provided enough detailed information above. | 2023-03-05T07:23:09 |
streamlink/streamlink | 5,222 | streamlink__streamlink-5222 | [
"5219"
] | 236116bbc9fb463aeb25e05625753827735f57e2 | diff --git a/src/streamlink/plugins/steam.py b/src/streamlink/plugins/steam.py
--- a/src/streamlink/plugins/steam.py
+++ b/src/streamlink/plugins/steam.py
@@ -137,7 +137,7 @@ def dologin(self, email, password, emailauth="", emailsteamid="", captchagid="-1
if not captcha_text:
return False
else:
- # If the user must enter the code that was emailed to them
+ # Whether the user must enter the code that was emailed to them
if resp.get("emailauth_needed"):
if emailauth:
raise SteamLoginFailed("Email auth key error")
@@ -148,7 +148,7 @@ def dologin(self, email, password, emailauth="", emailsteamid="", captchagid="-1
if not emailauth:
return False
- # If the user must enter a two factor auth code
+ # Whether the user must enter a two-factor auth code
if resp.get("requires_twofactor"):
try:
twofactorcode = self.input_ask("Two factor auth code required")
@@ -186,12 +186,11 @@ def _get_broadcast_stream(self, steamid, viewertoken=0, sessionid=None):
schema=validate.Schema(
validate.parse_json(),
{
- "success": validate.any("ready", "unavailable", "waiting", "waiting_to_start", "waiting_for_start"),
- "retry": int,
- "broadcastid": validate.any(str, int),
- validate.optional("url"): validate.url(),
- validate.optional("viewertoken"): str,
+ "success": str,
+ "url": validate.url(),
+ "cdn_auth_url_parameters": validate.any(str, None),
},
+ validate.union_get("success", "url", "cdn_auth_url_parameters"),
),
)
@@ -232,20 +231,17 @@ def _get_streams(self):
res = self.session.http.get(self.url) # get the page to set some cookies
sessionid = res.cookies.get("sessionid")
- streamdata = None
- while streamdata is None or streamdata["success"] in ("waiting", "waiting_for_start"):
- streamdata = self._get_broadcast_stream(steamid, sessionid=sessionid)
+ success, url, cdn_auth = self._get_broadcast_stream(steamid, sessionid=sessionid)
+ if success != "ready":
+ log.error("This stream is currently unavailable")
+ return
- if streamdata["success"] == "ready":
- return DASHStream.parse_manifest(self.session, streamdata["url"])
+ # CDN auth data is only required at certain times of the day, and it makes
+ # segment requests fail if missing (the DASH manifest still works regardless).
+ # Remove leading ampersand and pass the params as a string, to avoid URL quoting.
+ params = re.sub(r"^&", "", cdn_auth) if cdn_auth else None
- if streamdata["success"] == "unavailable":
- log.error("This stream is currently unavailable")
- return
-
- r = streamdata["retry"] / 1000.0
- log.info(f"Waiting for stream, will retry again in {r:.1f} seconds...")
- time.sleep(r)
+ return DASHStream.parse_manifest(self.session, url, params=params)
__plugin__ = SteamBroadcastPlugin
| plugins.steam: DASH segment requests missing token query-string parameter, sometimes leading to 403 error
### Checklist
- [X] This is a plugin issue and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest build from the master branch
### Description
Segment requests on the webplayer on steamcommunity.com always include a `token` query-string parameter with the user's IP and an hmac. The Streamlink plugin does not include that, and requests can fail with a 403, but only sometimes.
Webplayer request with `token`
```
https://steambroadcast.akamaized.net/broadcast/76561199177801954/3374786983679056684/segment/video/1/init/?broadcast_origin=ext2-mad1.steamserver.net&token=ip=REDACTED~exp=1678046973~acl=/broadcast/76561199177801954/3374786983679056684/*~hmac=98dabb3a60f2656bb9224234df1bffaa6d44e9b9
```
### Debug log
```text
$ streamlink -l debug 'https://steamcommunity.com/broadcast/watch/76561199177801954' best
[cli][debug] OS: Linux-6.1.12-1-git-x86_64-with-glibc2.37
[cli][debug] Python: 3.11.1
[cli][debug] Streamlink: 5.3.1+12.g41108844
[cli][debug] Dependencies:
[cli][debug] certifi: 2022.12.7
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.2
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.17
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.28.2
[cli][debug] urllib3: 1.26.14
[cli][debug] websocket-client: 1.5.1
[cli][debug] Arguments:
[cli][debug] url=https://steamcommunity.com/broadcast/watch/76561199177801954
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --player=mpv
[plugins.steam][debug] Restored cookies: REDACTED
[cli][info] Found matching plugin steam for URL https://steamcommunity.com/broadcast/watch/76561199177801954
[plugins.steam][debug] Getting broadcast stream: sessionid=REDACTED
[utils.l10n][debug] Language code: en_US
[stream.dash][debug] Available languages for DASH audio streams: und (using: und)
[cli][info] Available streams: 360p (worst), 480p, 720p (best)
[cli][info] Opening stream: 720p (dash)
[cli][info] Starting player: mpv
[stream.dash][debug] Opening DASH reader for: ('1', 'video', '1') - video/mp4
[stream.dash][debug] Opening DASH reader for: ('1', 'audio', '1') - audio/mp4
[stream.dash_manifest][debug] Generating segment numbers for dynamic playlist: ('1', 'video', '1')
[stream.dash_manifest][debug] Stream start: 2023-03-05 09:15:06+00:00
[stream.dash_manifest][debug] Current time: 2023-03-05 17:07:21.152912+00:00
[stream.dash_manifest][debug] Availability: 2023-03-05 17:07:14+00:00
[stream.dash_manifest][debug] presentationTimeOffset: 0:00:00; suggestedPresentationDelay: 0:00:03; minBufferTime: 0:00:03
[stream.dash_manifest][debug] segmentDuration: 2.0; segmentStart: 1; segmentOffset: 14165 (28329.152912s)
[stream.dash_manifest][debug] Generating segment numbers for dynamic playlist: ('1', 'audio', '1')
[stream.dash_manifest][debug] Stream start: 2023-03-05 09:15:06+00:00
[stream.dash_manifest][debug] Current time: 2023-03-05 17:07:21.155688+00:00
[stream.dash_manifest][debug] Availability: 2023-03-05 17:07:14+00:00
[stream.dash_manifest][debug] presentationTimeOffset: 0:00:00; suggestedPresentationDelay: 0:00:03; minBufferTime: 0:00:03
[stream.dash_manifest][debug] segmentDuration: 2.0; segmentStart: 1; segmentOffset: 14165 (28329.155688s)
[stream.dash][error] Failed to open video/mp4 segment https://steambroadcast.akamaized.net/broadcast/76561199177801954/3374786983679056684/segment/video/1/init/?broadcast_origin=ext2-mad1.steamserver.net: Unable to open URL: https://steambroadcast.akamaized.net/broadcast/76561199177801954/3374786983679056684/segment/video/1/init/?broadcast_origin=ext2-mad1.steamserver.net (403 Client Error: Forbidden for url: https://steambroadcast.akamaized.net/broadcast/76561199177801954/3374786983679056684/segment/video/1/init/?broadcast_origin=ext2-mad1.steamserver.net)
[stream.ffmpegmux][debug] ffmpeg version n5.1.2 Copyright (c) 2000-2022 the FFmpeg developers
[stream.ffmpegmux][debug] built with gcc 12.2.0 (GCC)
[stream.ffmpegmux][debug] configuration: --prefix=/usr --disable-debug --disable-static --disable-stripping --enable-amf --enable-avisynth --enable-cuda-llvm --enable-lto --enable-fontconfig --enable-gmp --enable-gnutls --enable-gpl --enable-ladspa --enable-libaom --enable-libass --enable-libbluray --enable-libbs2b --enable-libdav1d --enable-libdrm --enable-libfreetype --enable-libfribidi --enable-libgsm --enable-libiec61883 --enable-libjack --enable-libmfx --enable-libmodplug --enable-libmp3lame --enable-libopencore_amrnb --enable-libopencore_amrwb --enable-libopenjpeg --enable-libopus --enable-libpulse --enable-librav1e --enable-librsvg --enable-libsoxr --enable-libspeex --enable-libsrt --enable-libssh --enable-libsvtav1 --enable-libtheora --enable-libv4l2 --enable-libvidstab --enable-libvmaf --enable-libvorbis --enable-libvpx --enable-libwebp --enable-libx264 --enable-libx265 --enable-libxcb --enable-libxml2 --enable-libxvid --enable-libzimg --enable-nvdec --enable-nvenc --enable-opencl --enable-opengl --enable-shared --enable-version3 --enable-vulkan
[stream.ffmpegmux][debug] libavutil 57. 28.100 / 57. 28.100
[stream.ffmpegmux][debug] libavcodec 59. 37.100 / 59. 37.100
[stream.ffmpegmux][debug] libavformat 59. 27.100 / 59. 27.100
[stream.ffmpegmux][debug] libavdevice 59. 7.100 / 59. 7.100
[stream.ffmpegmux][debug] libavfilter 8. 44.100 / 8. 44.100
[stream.ffmpegmux][debug] libswscale 6. 7.100 / 6. 7.100
[stream.ffmpegmux][debug] libswresample 4. 7.100 / 4. 7.100
[stream.ffmpegmux][debug] libpostproc 56. 6.100 / 56. 6.100
[utils.named_pipe][info] Creating pipe streamlinkpipe-574031-1-7242
[utils.named_pipe][info] Creating pipe streamlinkpipe-574031-2-2694
[stream.ffmpegmux][debug] ffmpeg command: /usr/bin/ffmpeg -nostats -y -i /tmp/streamlinkpipe-574031-1-7242 -i /tmp/streamlinkpipe-574031-2-2694 -c:v copy -c:a copy -copyts -f matroska pipe:1
[stream.ffmpegmux][debug] Starting copy to pipe: /tmp/streamlinkpipe-574031-1-7242
[stream.ffmpegmux][debug] Starting copy to pipe: /tmp/streamlinkpipe-574031-2-2694
[cli][debug] Pre-buffering 8192 bytes
[stream.dash][error] Failed to open video/mp4 segment https://steambroadcast.akamaized.net/broadcast/76561199177801954/3374786983679056684/segment/video/1/init/?broadcast_origin=ext2-mad1.steamserver.net: Unable to open URL: https://steambroadcast.akamaized.net/broadcast/76561199177801954/3374786983679056684/segment/video/1/init/?broadcast_origin=ext2-mad1.steamserver.net (403 Client Error: Forbidden for url: https://steambroadcast.akamaized.net/broadcast/76561199177801954/3374786983679056684/segment/video/1/init/?broadcast_origin=ext2-mad1.steamserver.net)
...
```
| 2023-03-06T19:10:23 |
||
streamlink/streamlink | 5,233 | streamlink__streamlink-5233 | [
"5217"
] | 49682ddda44acfa27243e026839f738f81ddd752 | diff --git a/src/streamlink/stream/dash_manifest.py b/src/streamlink/stream/dash_manifest.py
--- a/src/streamlink/stream/dash_manifest.py
+++ b/src/streamlink/stream/dash_manifest.py
@@ -736,14 +736,12 @@ def segment_numbers(self) -> Iterator[Tuple[int, datetime.datetime]]:
buffer_time = self.root.minBufferTime
# Segment number
- # To reduce unnecessary delay, start with the next/upcoming segment: +1
seconds_offset = (since_start - suggested_delay - buffer_time).total_seconds()
- number_offset = max(0, int(seconds_offset / self.duration_seconds) + 1)
+ number_offset = max(0, int(seconds_offset / self.duration_seconds))
number_iter = count(self.startNumber + number_offset)
# Segment availability time
- # The availability time marks the segment's beginning: -1
- available_offset = datetime.timedelta(seconds=max(0, number_offset - 1) * self.duration_seconds)
+ available_offset = datetime.timedelta(seconds=number_offset * self.duration_seconds)
available_start = self.period.availabilityStartTime + available_offset
available_iter = count_dt(
available_start,
| diff --git a/tests/stream/test_dash_parser.py b/tests/stream/test_dash_parser.py
--- a/tests/stream/test_dash_parser.py
+++ b/tests/stream/test_dash_parser.py
@@ -147,15 +147,15 @@ def test_segments_dynamic_number(self):
datetime.datetime(2000, 1, 1, 0, 1, 30, tzinfo=UTC),
),
(
- "http://test/hd-5_000000794.mp4",
+ "http://test/hd-5_000000793.mp4",
datetime.datetime(2000, 1, 1, 0, 59, 15, tzinfo=UTC),
),
(
- "http://test/hd-5_000000795.mp4",
+ "http://test/hd-5_000000794.mp4",
datetime.datetime(2000, 1, 1, 0, 59, 20, tzinfo=UTC),
),
(
- "http://test/hd-5_000000796.mp4",
+ "http://test/hd-5_000000795.mp4",
datetime.datetime(2000, 1, 1, 0, 59, 25, tzinfo=UTC),
),
]
| stream.dash: fix dynamic timeline-less streams
- Fix segment number iterator and segment availability-time iterator for dynamic timeline-less DASH streams by calculating the right segment number offset and basing the segment availability-time on the segment number, and not just on the current time alone
- Set a default value of 0 seconds for presentationTimeOffset
- Ensure that segment durations are known
- Never calculate negative segment number offsets
- Reduce unnecessary delay by starting with the upcoming segment in the generated timeline to be as close to the live-edge as possible (with minBufferTime and presentation offset+delay in mind)
- Add debug log output
- Update MPD manifest test fixture with better time data
Next:
- Consider removing the default value of suggestedPresentationDelay, as it seems to be a relict of Streamlink's initial DASH implementation
- Also take a look at properly synchronizing the generated timeline between multiple substreams, so that the correct segment numbers always get calculated in all threads
- Avoid interweaving debug log and make it flush per-thread
----
Closes #4607
Not 100% sure whether this will fix #4607, but the data in that thread is not enough to confirm that, so let's close it. Since this PR adds more debug log messages with actual timestamps, new issue threads can be opened if there's still a problem with DASH streams of that particular kind.
At least this PR fixes all issues with DASH streams from the steam plugin, and the correct segment numbers at the correct times should be queued and requested by the worker and writer threads respectively.
| Example debug log from a random steamcommunity.com stream. No random failed segment requests anymore due to invalid segment numbers / availability times. Tested actual streams dozens of times.
Default value of `suggestedPresentationDelay` temporarily set to 0, so only the manifest's `minBufferTime` adds delay. The log shows that in the wait times, where the third segment requires a wait time of 0.7s while the first two are accessible immediately (segment duration = 2, minBufferTime = 3 -> 2 buffered segments). The remaining ones after that are queued properly.
```
...
[cli][info] Available streams: 360p (worst), 480p, 720p (best)
[cli][info] Opening stream: 720p (dash)
[cli][info] Starting player: mpv
[stream.dash][debug] Opening DASH reader for: ('1', 'video', '1') - video/mp4
[stream.dash][debug] Opening DASH reader for: ('1', 'audio', '1') - audio/mp4
[stream.dash_manifest][debug] Generating segment numbers for dynamic playlist: ('1', 'video', '1')
[stream.dash_manifest][debug] Stream start: 2023-03-03 17:11:53+00:00
[stream.dash_manifest][debug] Current time: 2023-03-05 07:27:40.102945+00:00
[stream.dash_manifest][debug] Availability: 2023-03-05 07:27:37+00:00
[stream.dash_manifest][debug] presentationTimeOffset: 0:00:00; suggestedPresentationDelay: 0:00:00; minBufferTime: 0:00:03
[stream.dash_manifest][debug] segmentDuration: 2.0; segmentStart: 1; segmentOffset: 68873 (137744.102945s)
[stream.dash_manifest][debug] Generating segment numbers for dynamic playlist: ('1', 'audio', '1')
[stream.dash_manifest][debug] Stream start: 2023-03-03 17:11:53+00:00
[stream.dash_manifest][debug] Current time: 2023-03-05 07:27:40.104530+00:00
[stream.dash_manifest][debug] Availability: 2023-03-05 07:27:37+00:00
[stream.dash_manifest][debug] presentationTimeOffset: 0:00:00; suggestedPresentationDelay: 0:00:00; minBufferTime: 0:00:03
[stream.dash_manifest][debug] segmentDuration: 2.0; segmentStart: 1; segmentOffset: 68873 (137744.10453s)
[stream.dash][debug] Download of video/mp4 segment: init complete
[stream.ffmpegmux][debug] ffmpeg version n5.1.2 Copyright (c) 2000-2022 the FFmpeg developers
...
[utils.named_pipe][info] Creating pipe streamlinkpipe-545850-1-930
[utils.named_pipe][info] Creating pipe streamlinkpipe-545850-2-8275
[stream.ffmpegmux][debug] ffmpeg command: /usr/bin/ffmpeg -nostats -y -i /tmp/streamlinkpipe-545850-1-930 -i /tmp/streamlinkpipe-545850-2-8275 -c:v copy -c:a copy -copyts -f matroska pipe:1
[stream.ffmpegmux][debug] Starting copy to pipe: /tmp/streamlinkpipe-545850-1-930
[stream.ffmpegmux][debug] Starting copy to pipe: /tmp/streamlinkpipe-545850-2-8275
[cli][debug] Pre-buffering 8192 bytes
[stream.dash][debug] Download of audio/mp4 segment: init complete
[stream.dash][debug] Download of video/mp4 segment: 68874 complete
[stream.dash][debug] Download of audio/mp4 segment: 68874 complete
[stream.dash][debug] Waiting for video/mp4 segment: 68876 (0.7s)
[stream.dash][debug] Download of video/mp4 segment: 68875 complete
[cli.output][debug] Opening subprocess: mpv --force-media-title=https://steamcommunity.com/broadcast/watch/76561199177801954 -
[stream.dash][debug] Download of audio/mp4 segment: 68875 complete
[stream.dash][debug] Waiting for audio/mp4 segment: 68876 (0.7s)
[cli][debug] Writing stream to output
[stream.dash][debug] Waiting for audio/mp4 segment: 68877 (2.0s)
[stream.dash][debug] Download of audio/mp4 segment: 68876 complete
[stream.dash][debug] Waiting for video/mp4 segment: 68877 (1.9s)
[stream.dash][debug] Download of video/mp4 segment: 68876 complete
[stream.dash][debug] Waiting for audio/mp4 segment: 68878 (1.9s)
[stream.dash][debug] Download of audio/mp4 segment: 68877 complete
[cli][info] Player closed
```
Got confused for a sec. There's a different issue with the Steam plugin where I've tested the DASH streams for this issue here, but that's unrelated to DASH itself. | 2023-03-10T00:25:15 |
streamlink/streamlink | 5,255 | streamlink__streamlink-5255 | [
"5254"
] | 98355078f1a0386b64b1532b1f8a6a4a820af6e0 | diff --git a/src/streamlink/plugins/twitcasting.py b/src/streamlink/plugins/twitcasting.py
--- a/src/streamlink/plugins/twitcasting.py
+++ b/src/streamlink/plugins/twitcasting.py
@@ -7,11 +7,14 @@
import hashlib
import logging
import re
+import sys
+from time import time
from streamlink.buffers import RingBuffer
-from streamlink.plugin import Plugin, PluginError, pluginargument, pluginmatcher
+from streamlink.plugin import Plugin, pluginargument, pluginmatcher
from streamlink.plugin.api import validate
from streamlink.plugin.api.websocket import WebsocketClient
+from streamlink.stream.hls import HLSStream
from streamlink.stream.stream import Stream, StreamIO
from streamlink.utils.url import update_qsd
@@ -29,66 +32,103 @@
help="Password for private Twitcasting streams.",
)
class TwitCasting(Plugin):
- _STREAM_INFO_URL = "https://twitcasting.tv/streamserver.php?target={channel}&mode=client"
- _STREAM_REAL_URL = "{proto}://{host}/ws.app/stream/{movie_id}/fmp4/bd/1/1500?mode={mode}"
-
- _STREAM_INFO_SCHEMA = validate.Schema({
- validate.optional("movie"): {
- "id": int,
- "live": bool,
- },
- validate.optional("fmp4"): {
- "host": str,
- "proto": str,
- "source": bool,
- "mobilesource": bool,
- },
- })
-
- def __init__(self, *args, **kwargs):
- super().__init__(*args, **kwargs)
- self.channel = self.match.group("channel")
+ _URL_API_STREAMSERVER = "https://twitcasting.tv/streamserver.php"
+ _URL_STREAM_HLS = "https://{host}/{channel}/metastream.m3u8"
+ _URL_STREAM_WEBSOCKET = "wss://{host}/ws.app/stream/{id}/fmp4/bd/1/1500?mode={mode}"
+
+ _STREAM_HOST_DEFAULT = "twitcasting.tv"
+
+ _WEBSOCKET_MODES = {
+ "main": "source",
+ "mobilesource": "mobilesource",
+ "base": None,
+ }
+
+ # prefer websocket streams over HLS streams due to latency reasons
+ _WEIGHTS = {
+ "main": sys.maxsize,
+ "mobilesource": sys.maxsize - 1,
+ "base": sys.maxsize - 2,
+ }
+
+ @classmethod
+ def stream_weight(cls, stream):
+ return (cls._WEIGHTS[stream], "none") if stream in cls._WEIGHTS else super().stream_weight(stream)
+
+ def _api_query_streamserver(self):
+ return self.session.http.get(
+ self._URL_API_STREAMSERVER,
+ params={
+ "target": self.match["channel"],
+ "mode": "client",
+ },
+ schema=validate.Schema(
+ validate.parse_json(),
+ {
+ validate.optional("movie"): {
+ "id": int,
+ "live": bool,
+ },
+ # ignore llfmp4 websocket streams, as those seem to cause video/audio desync
+ validate.optional("fmp4"): {
+ "proto": str,
+ "host": str,
+ "source": bool,
+ "mobilesource": bool,
+ },
+ # ignore the "dvr" HLS URL, as it results in a 403 response
+ validate.optional("hls"): {
+ "host": str,
+ "proto": str,
+ "source": bool,
+ },
+ },
+ validate.union_get("movie", "fmp4", "hls"),
+ ),
+ )
- def _get_streams(self):
- stream_info = self._get_stream_info()
- log.debug(f"Live stream info: {stream_info}")
+ def _get_streams_hls(self, data):
+ host = data.get("host") or self._STREAM_HOST_DEFAULT
+ url = self._URL_STREAM_HLS.format(host=host, channel=self.match["channel"])
+ params = {"__n": int(time() * 1000)}
- if not stream_info.get("movie") or not stream_info["movie"]["live"]:
- raise PluginError("The live stream is offline")
+ streams = [params]
+ if data.get("source"):
+ streams.append({"mode": "source", **params})
- if not stream_info.get("fmp4"):
- raise PluginError("Login required")
+ for params in streams:
+ yield from HLSStream.parse_variant_playlist(self.session, url, params=params).items()
- # Keys are already validated by schema above
- proto = stream_info["fmp4"]["proto"]
- host = stream_info["fmp4"]["host"]
- movie_id = stream_info["movie"]["id"]
+ def _get_streams_websocket(self, data):
+ host = data.get("host") or self._STREAM_HOST_DEFAULT
+ password = self.options.get("password")
- if stream_info["fmp4"]["source"]:
- mode = "main" # High quality
- elif stream_info["fmp4"]["mobilesource"]:
- mode = "mobilesource" # Medium quality
- else:
- mode = "base" # Low quality
+ for mode, prop in self._WEBSOCKET_MODES.items():
+ if prop is not None and not data.get(prop):
+ continue
- if (proto == "") or (host == "") or (not movie_id):
- raise PluginError(f"No stream available for user {self.channel}")
+ url = self._URL_STREAM_WEBSOCKET.format(host=host, id=self.id, mode=mode)
+ if password is not None:
+ password_hash = hashlib.md5(password.encode()).hexdigest()
+ url = update_qsd(url, {"word": password_hash})
- real_stream_url = self._STREAM_REAL_URL.format(proto=proto, host=host, movie_id=movie_id, mode=mode)
+ yield mode, TwitCastingStream(self.session, url)
- password = self.options.get("password")
- if password is not None:
- password_hash = hashlib.md5(password.encode()).hexdigest()
- real_stream_url = update_qsd(real_stream_url, {"word": password_hash})
-
- log.debug(f"Real stream url: {real_stream_url}")
+ def _get_streams(self):
+ movie, websocket, hls = self._api_query_streamserver()
+ if not movie or not movie.get("id") or not movie.get("live"):
+ log.error(f"No live stream available for user {self.match['channel']}")
+ return
+ if not websocket and not hls:
+ log.error("Unsupported stream type")
+ return
- return {mode: TwitCastingStream(session=self.session, url=real_stream_url)}
+ self.id = movie.get("id")
- def _get_stream_info(self):
- url = self._STREAM_INFO_URL.format(channel=self.channel)
- res = self.session.http.get(url)
- return self.session.http.json(res, schema=self._STREAM_INFO_SCHEMA)
+ if websocket:
+ yield from self._get_streams_websocket(websocket)
+ if hls:
+ yield from self._get_streams_hls(hls)
class TwitCastingWsClient(WebsocketClient):
| diff --git a/tests/plugins/test_twitcasting.py b/tests/plugins/test_twitcasting.py
--- a/tests/plugins/test_twitcasting.py
+++ b/tests/plugins/test_twitcasting.py
@@ -6,6 +6,5 @@ class TestPluginCanHandleUrlTwitCasting(PluginCanHandleUrl):
__plugin__ = TwitCasting
should_match = [
- "https://twitcasting.tv/c:kk1992kkkk",
- "https://twitcasting.tv/icchy8591/movie/566593738",
+ "https://twitcasting.tv/twitcasting_jp",
]
| plugins.twitcasting: Doesn't download the stream, incorrectly prints "[cli][error] Login required"
### Checklist
- [X] This is a plugin issue and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest stable release
### Description
Previously [we have made an assumption](https://github.com/streamlink/streamlink/issues/4281#issuecomment-1008870858) that if there is no `fmp4` key in the `streamserver.php` json response, then the stream required login. However, looks like it's not (entirely) true. There is a stream currently going on at https://twitcasting.tv/twitcasting_jp and `streamserver.php` returns the same looking json as in that previous issue:
```json
{
"movie": {
"id": 762209471,
"live": true
},
"hls": {
"host": "twitcasting.tv",
"proto": "https",
"source": true
}
}
```
but the stream doesn't show the "You must be logged in to watch" message when viewed in Chrome's Incognito, the stream actually plays just fine.
Checking the browser network requests, it requests `https://twitcasting.tv/twitcasting_jp/metastream.m3u8?mode=source&__n=1679193002006`, receiving back something like:
```
#EXTM3U
#EXT-X-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=3000000,CODECS="mp4a.40.2,avc1.4D401E"
https://202-218-193-225.twitcasting.tv/mpegts/762209471-2f3e7000786d37eb/ts-source-mp4.m3u8
```
and then it keeps periodically requesting `https://202-218-193-225.twitcasting.tv/mpegts/762209471-2f3e7000786d37eb/ts-source-mp4.m3u8`, getting something like:
```
#EXTM3U
#EXT-X-ALLOW-CACHE:NO
#EXT-X-VERSION:6
#EXT-X-MEDIA-SEQUENCE:1688
#EXT-X-TARGETDURATION:2
#EXT-X-KEY:METHOD=AES-128,URI="/livelicense.php?u=%2Fmpegts%2F762209471-2f3e7000786d37eb%2Fts-source-0",IV=0xc2f5b1e309ea3434736214b2b0bfe7b1
#EXT-X-MAP:URI="/mpegts/762209471-2f3e7000786d37eb/ts-source-0.mp4"
#EXTINF:2.002,
/mpegts/762209471-2f3e7000786d37eb/ts-source-1688.mp4
#EXTINF:2.002,
/mpegts/762209471-2f3e7000786d37eb/ts-source-1689.mp4
#EXTINF:2.002,
/mpegts/762209471-2f3e7000786d37eb/ts-source-1690.mp4
#EXTINF:2.002,
/mpegts/762209471-2f3e7000786d37eb/ts-source-1691.mp4
#EXTINF:2.002,
/mpegts/762209471-2f3e7000786d37eb/ts-source-1692.mp4
#EXTINF:2.002,
/mpegts/762209471-2f3e7000786d37eb/ts-source-1693.mp4
```
getting `livelicense.php`, `ts-source-0.mp4` and downloading the corresponding `ts-source-*.mp4` files.
It seems to be a pre-recorded stream that Twitcasting is playing, so perhaps that's why it's such an outlier and doesn't use WebSocket to communicate.
### Debug log
```text
$ streamlink --loglevel=debug 'https://twitcasting.tv/twitcasting_jp'
[cli][debug] OS: Linux-5.10.0-20-amd64-x86_64-with-glibc2.31
[cli][debug] Python: 3.9.2
[cli][debug] Streamlink: 5.3.1
[cli][debug] Dependencies:
[cli][debug] certifi: 2020.12.5
[cli][debug] isodate: 0.6.0
[cli][debug] lxml: 4.7.1
[cli][debug] pycountry: 20.7.3
[cli][debug] pycryptodome: 3.10.1
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.28.0
[cli][debug] urllib3: 1.26.3
[cli][debug] websocket-client: 1.2.3
[cli][debug] Arguments:
[cli][debug] url=https://twitcasting.tv/twitcasting_jp
[cli][debug] --loglevel=debug
[cli][info] Found matching plugin twitcasting for URL https://twitcasting.tv/twitcasting_jp
[plugins.twitcasting][debug] Live stream info: {'movie': {'id': 762209471, 'live': True}}
error: Login required
```
| 2023-03-19T06:27:03 |
|
streamlink/streamlink | 5,262 | streamlink__streamlink-5262 | [
"5257"
] | 45e515eb5a57ea047685aa1088777cce313db09c | diff --git a/src/streamlink/stream/dash.py b/src/streamlink/stream/dash.py
--- a/src/streamlink/stream/dash.py
+++ b/src/streamlink/stream/dash.py
@@ -3,6 +3,7 @@
import logging
from collections import defaultdict
from contextlib import contextmanager, suppress
+from datetime import datetime
from time import time
from typing import Any, Dict, List, Optional, Tuple
from urllib.parse import urlparse, urlunparse
@@ -15,6 +16,7 @@
from streamlink.stream.stream import Stream
from streamlink.utils.l10n import Language
from streamlink.utils.parse import parse_xml
+from streamlink.utils.times import now
log = logging.getLogger(__name__)
@@ -108,7 +110,12 @@ def iter_segments(self):
if not representation:
continue
- for segment in representation.segments(init=init):
+ iter_segments = representation.segments(
+ init=init,
+ # sync initial timeline generation between audio and video threads
+ timestamp=self.reader.timestamp if init else None,
+ )
+ for segment in iter_segments:
if self.closed:
break
yield segment
@@ -163,10 +170,18 @@ class DASHStreamReader(SegmentedStreamReader):
writer: "DASHStreamWriter"
stream: "DASHStream"
- def __init__(self, stream: "DASHStream", representation: Representation, *args, **kwargs):
+ def __init__(
+ self,
+ stream: "DASHStream",
+ representation: Representation,
+ timestamp: datetime,
+ *args,
+ **kwargs,
+ ):
super().__init__(stream, *args, **kwargs)
self.ident = representation.ident
self.mime_type = representation.mimeType
+ self.timestamp = timestamp
class DASHStream(Stream):
@@ -357,13 +372,15 @@ def open(self):
video, audio = None, None
rep_video, rep_audio = self.video_representation, self.audio_representation
+ timestamp = now()
+
if rep_video:
- video = DASHStreamReader(self, rep_video)
+ video = DASHStreamReader(self, rep_video, timestamp)
log.debug(f"Opening DASH reader for: {rep_video.ident!r} - {rep_video.mimeType}")
video.open()
if rep_audio:
- audio = DASHStreamReader(self, rep_audio)
+ audio = DASHStreamReader(self, rep_audio, timestamp)
log.debug(f"Opening DASH reader for: {rep_audio.ident!r} - {rep_audio.mimeType}")
audio.open()
diff --git a/src/streamlink/stream/dash_manifest.py b/src/streamlink/stream/dash_manifest.py
--- a/src/streamlink/stream/dash_manifest.py
+++ b/src/streamlink/stream/dash_manifest.py
@@ -635,13 +635,14 @@ def lang(self):
def bandwidth_rounded(self) -> float:
return round(self.bandwidth, 1 - int(math.log10(self.bandwidth)))
- def segments(self, **kwargs) -> Iterator[Segment]:
+ def segments(self, timestamp: Optional[datetime] = None, **kwargs) -> Iterator[Segment]:
"""
Segments are yielded when they are available
Segments appear on a timeline, for dynamic content they are only available at a certain time
and sometimes for a limited time. For static content they are all available at the same time.
+ :param timestamp: Optional initial timestamp for syncing timelines of multiple substreams
:param kwargs: extra args to pass to the segment template
:return: yields Segments
"""
@@ -654,6 +655,7 @@ def segments(self, **kwargs) -> Iterator[Segment]:
yield from segmentTemplate.segments(
self.ident,
self.base_url,
+ timestamp=timestamp,
RepresentationID=self.id,
Bandwidth=int(self.bandwidth * 1000),
**kwargs,
@@ -790,7 +792,13 @@ def __init__(self, *args, **kwargs) -> None:
parser=MPDParsers.segment_template,
)
- def segments(self, ident: TTimelineIdent, base_url: str, **kwargs) -> Iterator[Segment]:
+ def segments(
+ self,
+ ident: TTimelineIdent,
+ base_url: str,
+ timestamp: Optional[datetime] = None,
+ **kwargs,
+ ) -> Iterator[Segment]:
if kwargs.pop("init", True): # pragma: no branch
init_url = self.format_initialization(base_url, **kwargs)
if init_url: # pragma: no branch
@@ -803,7 +811,7 @@ def segments(self, ident: TTimelineIdent, base_url: str, **kwargs) -> Iterator[S
content=False,
byterange=None,
)
- for media_url, number, available_at in self.format_media(ident, base_url, **kwargs):
+ for media_url, number, available_at in self.format_media(ident, base_url, timestamp=timestamp, **kwargs):
yield Segment(
url=media_url,
number=number,
@@ -818,7 +826,7 @@ def segments(self, ident: TTimelineIdent, base_url: str, **kwargs) -> Iterator[S
def make_url(base_url: str, url: str) -> str:
return BaseURL.join(base_url, url)
- def segment_numbers(self) -> Iterator[Tuple[int, datetime]]:
+ def segment_numbers(self, timestamp: Optional[datetime] = None) -> Iterator[Tuple[int, datetime]]:
"""
yield the segment number and when it will be available.
@@ -845,7 +853,7 @@ def segment_numbers(self) -> Iterator[Tuple[int, datetime]]:
else:
number_iter = count(self.startNumber)
else:
- current_time = now()
+ current_time = timestamp or now()
since_start = current_time - self.period.availabilityStartTime - self.presentationTimeOffset
suggested_delay = self.root.suggestedPresentationDelay
@@ -918,13 +926,19 @@ def format_initialization(self, base_url: str, **kwargs) -> Optional[str]:
if self.fmt_initialization is not None: # pragma: no branch
return self.make_url(base_url, self.fmt_initialization(**kwargs))
- def format_media(self, ident: TTimelineIdent, base_url: str, **kwargs) -> Iterator[Tuple[str, int, datetime]]:
+ def format_media(
+ self,
+ ident: TTimelineIdent,
+ base_url: str,
+ timestamp: Optional[datetime] = None,
+ **kwargs,
+ ) -> Iterator[Tuple[str, int, datetime]]:
if self.fmt_media is None: # pragma: no cover
return
if not self.segmentTimeline:
log.debug(f"Generating segment numbers for {self.root.type} playlist: {ident!r}")
- for number, available_at in self.segment_numbers():
+ for number, available_at in self.segment_numbers(timestamp=timestamp):
url = self.make_url(base_url, self.fmt_media(Number=number, **kwargs))
yield url, number, available_at
else:
| diff --git a/tests/stream/test_dash.py b/tests/stream/test_dash.py
--- a/tests/stream/test_dash.py
+++ b/tests/stream/test_dash.py
@@ -1,6 +1,8 @@
+from datetime import datetime, timezone
from typing import List
from unittest.mock import ANY, Mock, call
+import freezegun
import pytest
import requests_mock as rm
from lxml.etree import ParseError
@@ -13,6 +15,12 @@
from tests.resources import text, xml
[email protected]()
+def timestamp():
+ with freezegun.freeze_time("2000-01-01T00:00:00Z"):
+ yield datetime.now(timezone.utc)
+
+
class TestDASHStreamParseManifest:
@pytest.fixture(autouse=True)
def _response(self, request: pytest.FixtureRequest, requests_mock: rm.Mocker):
@@ -265,28 +273,29 @@ def muxer(self, monkeypatch: pytest.MonkeyPatch):
monkeypatch.setattr("streamlink.stream.dash.FFMPEGMuxer", muxer)
return muxer
- def test_stream_open_video_only(self, session: Streamlink, muxer: Mock, reader: Mock):
+ def test_stream_open_video_only(self, session: Streamlink, timestamp: datetime, muxer: Mock, reader: Mock):
rep_video = Mock(ident=(None, None, "1"), mimeType="video/mp4")
stream = DASHStream(session, Mock(), rep_video)
stream.open()
- assert reader.call_args_list == [call(stream, rep_video)]
- reader_video = reader(stream, rep_video)
+ assert reader.call_args_list == [call(stream, rep_video, timestamp)]
+ reader_video = reader(stream, rep_video, timestamp)
assert reader_video.open.called_once
assert muxer.call_args_list == []
- def test_stream_open_video_audio(self, session: Streamlink, muxer: Mock, reader: Mock):
+ def test_stream_open_video_audio(self, session: Streamlink, timestamp: datetime, muxer: Mock, reader: Mock):
rep_video = Mock(ident=(None, None, "1"), mimeType="video/mp4")
rep_audio = Mock(ident=(None, None, "2"), mimeType="audio/mp3", lang="en")
stream = DASHStream(session, Mock(), rep_video, rep_audio)
stream.open()
- assert reader.call_args_list == [call(stream, rep_video), call(stream, rep_audio)]
- reader_video = reader(stream, rep_video)
- reader_audio = reader(stream, rep_audio)
+ assert reader.call_args_list == [call(stream, rep_video, timestamp), call(stream, rep_audio, timestamp)]
+ reader_video = reader(stream, rep_video, timestamp)
+ reader_audio = reader(stream, rep_audio, timestamp)
assert reader_video.open.called_once
assert reader_audio.open.called_once
assert muxer.call_args_list == [call(session, reader_video, reader_audio, copyts=True)]
+ assert reader_video.timestamp is reader_audio.timestamp
class TestDASHStreamWorker:
@@ -339,15 +348,25 @@ def representation(self, mpd) -> Mock:
return mpd.periods[0].adaptationSets[0].representations[0]
@pytest.fixture()
- def worker(self, mpd):
- stream = Mock(mpd=mpd, period=0, args={})
- reader = Mock(stream=stream, ident=(None, None, "1"))
+ def worker(self, timestamp: datetime, mpd: Mock):
+ stream = Mock(
+ mpd=mpd,
+ period=0,
+ args={},
+ )
+ reader = Mock(
+ stream=stream,
+ ident=(None, None, "1"),
+ timestamp=timestamp,
+ )
worker = DASHStreamWorker(reader)
+
return worker
def test_dynamic_reload(
self,
monkeypatch: pytest.MonkeyPatch,
+ timestamp: datetime,
worker: DASHStreamWorker,
representation: Mock,
segments: List[Mock],
@@ -361,18 +380,19 @@ def test_dynamic_reload(
representation.segments.return_value = segments[:1]
assert next(segment_iter) is segments[0]
- assert representation.segments.call_args_list == [call(init=True)]
+ assert representation.segments.call_args_list == [call(init=True, timestamp=timestamp)]
assert not worker._wait.is_set()
representation.segments.reset_mock()
representation.segments.return_value = segments[1:]
assert [next(segment_iter), next(segment_iter)] == segments[1:]
- assert representation.segments.call_args_list == [call(), call(init=False)]
+ assert representation.segments.call_args_list == [call(), call(init=False, timestamp=None)]
assert not worker._wait.is_set()
def test_static(
self,
worker: DASHStreamWorker,
+ timestamp: datetime,
representation: Mock,
segments: List[Mock],
mpd: Mock,
@@ -382,7 +402,7 @@ def test_static(
representation.segments.return_value = segments
assert list(worker.iter_segments()) == segments
- assert representation.segments.call_args_list == [call(init=True)]
+ assert representation.segments.call_args_list == [call(init=True, timestamp=timestamp)]
assert worker._wait.is_set()
# Verify the fix for https://github.com/streamlink/streamlink/issues/2873
@@ -392,13 +412,14 @@ def test_static(
])
def test_static_refresh_wait(
self,
- duration: float,
+ timestamp: datetime,
mock_wait: Mock,
mock_time: Mock,
worker: DASHStreamWorker,
representation: Mock,
segments: List[Mock],
mpd: Mock,
+ duration: float,
):
mpd.dynamic = False
mpd.type = "static"
@@ -406,6 +427,6 @@ def test_static_refresh_wait(
representation.segments.return_value = segments
assert list(worker.iter_segments()) == segments
- assert representation.segments.call_args_list == [call(init=True)]
+ assert representation.segments.call_args_list == [call(init=True, timestamp=timestamp)]
assert mock_wait.call_args_list == [call(5)]
assert worker._wait.is_set()
diff --git a/tests/stream/test_dash_parser.py b/tests/stream/test_dash_parser.py
--- a/tests/stream/test_dash_parser.py
+++ b/tests/stream/test_dash_parser.py
@@ -1,6 +1,6 @@
import datetime
import itertools
-import unittest
+from contextlib import nullcontext
from operator import attrgetter
from unittest.mock import Mock
@@ -46,7 +46,7 @@ def test_availability(self):
assert segment.availability == "2000-01-02T03:04:05.123456Z / 2000-01-01T00:00:00.000000Z"
-class TestMPDParsers(unittest.TestCase):
+class TestMPDParsers:
def test_bool_str(self):
assert MPDParsers.bool_str("true")
assert MPDParsers.bool_str("TRUE")
@@ -89,9 +89,7 @@ def test_range(self):
MPDParsers.range("100")
-class TestMPDParser(unittest.TestCase):
- maxDiff = None
-
+class TestMPDParser:
def test_no_segment_list_or_template(self):
with xml("dash/test_no_segment_list_or_template.mpd") as mpd_xml:
mpd = MPD(mpd_xml, base_url="http://test/", url="http://test/manifest.mpd")
@@ -175,14 +173,26 @@ def test_segments_dynamic_time(self):
"http://test.se/video-time=1525450872000-2800000-0.m4s?z32=",
]
- def test_segments_dynamic_number(self):
- # access manifest one hour after its availabilityStartTime
+ # access manifest one hour after its availabilityStartTime
+ @pytest.mark.parametrize(("frozen_time", "timestamp"), [
+ pytest.param(
+ freeze_time("2000-01-01T01:00:00Z"),
+ None,
+ id="Without explicit timestamp",
+ ),
+ pytest.param(
+ nullcontext(),
+ datetime.datetime(2000, 1, 1, 1, 0, 0, 0, tzinfo=UTC),
+ id="With explicit timestamp",
+ ),
+ ])
+ def test_segments_dynamic_number(self, frozen_time, timestamp):
with xml("dash/test_segments_dynamic_number.mpd") as mpd_xml, \
- freeze_time("2000-01-01T01:00:00Z"):
+ frozen_time:
mpd = MPD(mpd_xml, base_url="http://test/", url="http://test/manifest.mpd")
stream_urls = [
(segment.url, segment.available_at)
- for segment in itertools.islice(mpd.periods[0].adaptationSets[0].representations[0].segments(), 4)
+ for segment in itertools.islice(mpd.periods[0].adaptationSets[0].representations[0].segments(timestamp), 4)
]
assert stream_urls == [
| stream.dash: generated timelines of substreams are not properly synchronized
### Checklist
- [X] This is a bug report and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed bug reports](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22bug%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest build from the master branch
### Description
The generated DASH timelines of audio and video streams are currently not properly synchronized.
This comes from the fact that the `DASHStreamReader` instances of the audio and video substreams do have their own worker threads where the timelines get generated by the parser. If the timeline depends on the current time (e.g. segment templates without a segment timeline), then this can lead to synchronization issues when the threads don't parse the manifest at the exact same time (which they never do). The window for desynchronization depends on each segment duration and the time it takes for the parser to parse the manifest and generate the timeline. It's a rather tiny window for failure, but the issue should still get fixed at some point.
The `DASHStreamReader` instance needs to receive a common timestamp in the `DASHStream.open()` call where the initial timeline needs to get built from.
### Debug log
```text
[stream.dash][debug] Opening DASH reader for: ('1', 'video', '1') - video/mp4
[stream.dash][debug] Opening DASH reader for: ('1', 'audio', '1') - audio/mp4
[stream.dash][debug] video/mp4 segment initialization: downloading (2023-03-20T04:12:05.000000Z / 2023-03-21T15:07:44.330582Z)
[stream.dash_manifest][debug] Generating segment numbers for dynamic playlist: ('1', 'video', '1')
[stream.dash_manifest][debug] Stream start: 2023-03-20 04:12:05+00:00
[stream.dash_manifest][debug] Current time: 2023-03-21 15:07:44.331717+00:00
[stream.dash_manifest][debug] Availability: 2023-03-21 15:07:37+00:00
[stream.dash_manifest][debug] presentationTimeOffset: 0:00:00; suggestedPresentationDelay: 0:00:03; minBufferTime: 0:00:03
[stream.dash_manifest][debug] segmentDuration: 2.0; segmentStart: 1; segmentOffset: 62866 (125733.331717s)
[stream.dash][debug] audio/mp4 segment initialization: downloading (2023-03-20T04:12:05.000000Z / 2023-03-21T15:07:44.332672Z)
[stream.dash_manifest][debug] Generating segment numbers for dynamic playlist: ('1', 'audio', '1')
[stream.dash_manifest][debug] Stream start: 2023-03-20 04:12:05+00:00
[stream.dash_manifest][debug] Current time: 2023-03-21 15:07:44.333485+00:00
[stream.dash_manifest][debug] Availability: 2023-03-21 15:07:37+00:00
[stream.dash_manifest][debug] presentationTimeOffset: 0:00:00; suggestedPresentationDelay: 0:00:03; minBufferTime: 0:00:03
[stream.dash_manifest][debug] segmentDuration: 2.0; segmentStart: 1; segmentOffset: 62866 (125733.333485s)
```
| 2023-03-27T11:52:04 |
|
streamlink/streamlink | 5,266 | streamlink__streamlink-5266 | [
"5130"
] | 781ef1fc92f215d0f3ec9a272fbe9f2cac122f08 | diff --git a/src/streamlink/plugins/indihometv.py b/src/streamlink/plugins/indihometv.py
new file mode 100644
--- /dev/null
+++ b/src/streamlink/plugins/indihometv.py
@@ -0,0 +1,48 @@
+"""
+$description Live TV channels and video on-demand service from IndiHome TV, owned by Telkom Indonesia.
+$url indihometv.com
+$type live, vod
+$region Indonesia
+"""
+
+import re
+
+from streamlink.plugin import Plugin, pluginmatcher
+from streamlink.plugin.api import validate
+from streamlink.stream.dash import DASHStream
+from streamlink.stream.hls import HLSStream
+
+
+@pluginmatcher(re.compile(r"https?://(?:www\.)?indihometv\.com/"))
+class IndiHomeTV(Plugin):
+ def _get_streams(self):
+ url = self.session.http.get(self.url, schema=validate.Schema(
+ validate.parse_html(),
+ validate.any(
+ validate.all(
+ validate.xml_xpath_string("""
+ .//script[contains(text(), 'laylist.m3u8') or contains(text(), 'manifest.mpd')][1]/text()
+ """),
+ str,
+ re.compile(r"""(?P<q>['"])(?P<url>https://.*?/(?:[Pp]laylist\.m3u8|manifest\.mpd).+?)(?P=q)"""),
+ validate.none_or_all(
+ validate.get("url"),
+ validate.url(),
+ ),
+ ),
+ validate.all(
+ validate.xml_xpath_string(".//video[@id='video-player'][1]/source[1]/@src"),
+ validate.none_or_all(
+ validate.url(),
+ ),
+ ),
+ ),
+ ))
+
+ if url and ".m3u8" in url:
+ return HLSStream.parse_variant_playlist(self.session, url)
+ elif url and ".mpd" in url:
+ return DASHStream.parse_manifest(self.session, url)
+
+
+__plugin__ = IndiHomeTV
diff --git a/src/streamlink/plugins/useetv.py b/src/streamlink/plugins/useetv.py
deleted file mode 100644
--- a/src/streamlink/plugins/useetv.py
+++ /dev/null
@@ -1,61 +0,0 @@
-"""
-$description Live TV channels and video on-demand service from UseeTV, owned by Telkom Indonesia.
-$url useetv.com
-$type live, vod
-"""
-
-import logging
-import re
-
-from streamlink.plugin import Plugin, pluginmatcher
-from streamlink.plugin.api import validate
-from streamlink.stream.dash import DASHStream
-from streamlink.stream.hls import HLSStream
-
-
-log = logging.getLogger(__name__)
-
-
-@pluginmatcher(re.compile(r"https?://(?:www\.)?useetv\.com/"))
-class UseeTV(Plugin):
- def _get_streams(self):
- root = self.session.http.get(self.url, schema=validate.Schema(validate.parse_html()))
-
- for needle, errormsg in (
- (
- "\"This service is not available in your Country\"",
- "The content is not available in your region",
- ),
- (
- "\"Silahkan login Menggunakan akun MyIndihome dan berlangganan minipack\"",
- "The content is not available without a subscription",
- ),
- ):
- if validate.Schema(validate.xml_xpath(".//script[contains(text(),$needle)]", needle=needle)).validate(root):
- log.error(errormsg)
- return
-
- url = validate.Schema(
- validate.any(
- validate.all(
- validate.xml_xpath_string("""
- .//script[contains(text(), 'laylist.m3u8') or contains(text(), 'manifest.mpd')][1]/text()
- """),
- str,
- re.compile(r"""(?P<q>['"])(?P<url>https://.*?/(?:[Pp]laylist\.m3u8|manifest\.mpd).+?)(?P=q)"""),
- validate.none_or_all(validate.get("url"), validate.url()),
- ),
- validate.all(
- validate.xml_xpath_string(".//video[@id='video-player']/source/@src"),
- validate.any(None, validate.url()),
- ),
- ),
- ).validate(root)
-
- if url and ".m3u8" in url:
- return HLSStream.parse_variant_playlist(self.session, url)
- elif url and ".mpd" in url:
- return DASHStream.parse_manifest(self.session, url)
-
-
-__plugin__ = UseeTV
| diff --git a/tests/plugins/test_indihometv.py b/tests/plugins/test_indihometv.py
new file mode 100644
--- /dev/null
+++ b/tests/plugins/test_indihometv.py
@@ -0,0 +1,12 @@
+from streamlink.plugins.indihometv import IndiHomeTV
+from tests.plugins import PluginCanHandleUrl
+
+
+class TestPluginCanHandleUrlIndiHomeTV(PluginCanHandleUrl):
+ __plugin__ = IndiHomeTV
+
+ should_match = [
+ "https://www.indihometv.com/livetv/seatoday",
+ "https://www.indihometv.com/livetv/transtv",
+ "https://www.indihometv.com/tvod/seatoday/1680109200/1680111000/18328552/voa-our-voice",
+ ]
diff --git a/tests/plugins/test_useetv.py b/tests/plugins/test_useetv.py
deleted file mode 100644
--- a/tests/plugins/test_useetv.py
+++ /dev/null
@@ -1,17 +0,0 @@
-from streamlink.plugins.useetv import UseeTV
-from tests.plugins import PluginCanHandleUrl
-
-
-class TestPluginCanHandleUrlUseeTV(PluginCanHandleUrl):
- __plugin__ = UseeTV
-
- should_match = [
- "http://useetv.com/any",
- "http://useetv.com/any/path",
- "http://www.useetv.com/any",
- "http://www.useetv.com/any/path",
- "https://useetv.com/any",
- "https://useetv.com/any/path",
- "https://www.useetv.com/any",
- "https://www.useetv.com/any/path",
- ]
| plugins.useetv: is now indihometv.com
### Checklist
- [X] This is a plugin issue and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest stable release
### Description
Maybe there will be a plugins update for this?
### Debug log
```text
streamlink -l trace https://useetv.com/livetv/transtv
[03:41:41.598371][cli][debug] OS: Linux-4.14.190-perf-g54a1ddf7276a-aarch64-with-libc
[03:41:41.598928][cli][debug] Python: 3.11.1
[03:41:41.599115][cli][debug] Streamlink: 5.2.1
[03:41:41.601963][cli][debug] Dependencies: [03:41:41.619678][cli][debug] certifi: 2022.12.7
[03:41:41.624379][cli][debug] isodate: 0.6.1
[03:41:41.627208][cli][debug] lxml: 4.9.2
[03:41:41.632570][cli][debug] pycountry: 22.3.5
[03:41:41.635104][cli][debug] pycryptodome: 3.16.0
[03:41:41.639277][cli][debug] PySocks: 1.7.1
[03:41:41.643652][cli][debug] requests: 2.28.1 [03:41:41.651013][cli][debug] urllib3: 1.26.13
[03:41:41.655050][cli][debug] websocket-client: 1.5.0 [03:41:41.656684][cli][debug] Arguments:
[03:41:41.657124][cli][debug] url=https://useetv.com/livetv/transtv
[03:41:41.657367][cli][debug] --loglevel=trace
[03:41:41.658296][cli][info] Found matching plugin useetv for URL https://useetv.com/livetv/transtv [03:41:43.705587][plugins.useetv][error] The content is not available in your region
error: No playable streams found on this URL: https://useetv.com/livetv/transtv
```
| Just change the url when using streamlink.
work for me. But i think this plugin need update. | 2023-03-30T16:00:52 |
streamlink/streamlink | 5,279 | streamlink__streamlink-5279 | [
"5277"
] | 8d0afbf908f948a14f6e6a554087b9e5da160068 | diff --git a/src/streamlink/plugins/nbcnews.py b/src/streamlink/plugins/nbcnews.py
deleted file mode 100644
--- a/src/streamlink/plugins/nbcnews.py
+++ /dev/null
@@ -1,90 +0,0 @@
-"""
-$description 24-hour world, US and local news channel, based in the United States of America.
-$url nbcnews.com
-$type live
-"""
-
-import logging
-import re
-
-from streamlink.plugin import Plugin, pluginmatcher
-from streamlink.plugin.api import validate
-from streamlink.stream.hls import HLSStream
-
-
-log = logging.getLogger(__name__)
-
-
-@pluginmatcher(re.compile(
- r"https?://(?:www\.)?nbcnews\.com/now",
-))
-class NBCNews(Plugin):
- URL_API = "https://api-leap.nbcsports.com/feeds/assets/{}?application=NBCNews&format=nbc-player&platform=desktop"
- URL_TOKEN = "https://tokens.playmakerservices.com/"
-
- title = "NBC News Now"
-
- def _get_streams(self):
- self.id = self.session.http.get(
- self.url,
- schema=validate.Schema(
- validate.parse_html(),
- validate.xml_xpath_string(".//script[@type='application/ld+json'][1]/text()"),
- validate.none_or_all(
- validate.parse_json(),
- {"embedUrl": validate.url()},
- validate.get("embedUrl"),
- validate.transform(lambda embed_url: embed_url.split("/")[-1]),
- ),
- ),
- )
- if self.id is None:
- return
- log.debug(f"API ID: {self.id}")
-
- stream = self.session.http.get(
- self.URL_API.format(self.id),
- schema=validate.Schema(
- validate.parse_json(),
- {
- "videoSources": [{
- "cdnSources": {
- "primary": [{
- "sourceUrl": validate.url(path=validate.endswith(".m3u8")),
- }],
- },
- }],
- },
- validate.get(("videoSources", 0, "cdnSources", "primary", 0, "sourceUrl")),
- ),
- )
-
- url = self.session.http.post(
- self.URL_TOKEN,
- json={
- "requestorId": "nbcnews",
- "pid": self.id,
- "application": "NBCSports",
- "version": "v1",
- "platform": "desktop",
- "token": "",
- "resourceId": "",
- "inPath": "false",
- "authenticationType": "unauth",
- "cdn": "akamai",
- "url": stream,
- },
- schema=validate.Schema(
- validate.parse_json(),
- {
- "akamai": [{
- "tokenizedUrl": validate.url(),
- }],
- },
- validate.get(("akamai", 0, "tokenizedUrl")),
- ),
- )
- return HLSStream.parse_variant_playlist(self.session, url)
-
-
-__plugin__ = NBCNews
| diff --git a/tests/plugins/test_nbcnews.py b/tests/plugins/test_nbcnews.py
deleted file mode 100644
--- a/tests/plugins/test_nbcnews.py
+++ /dev/null
@@ -1,16 +0,0 @@
-from streamlink.plugins.nbcnews import NBCNews
-from tests.plugins import PluginCanHandleUrl
-
-
-class TestPluginCanHandleUrlNBCNews(PluginCanHandleUrl):
- __plugin__ = NBCNews
-
- should_match = [
- "https://www.nbcnews.com/now/",
- "http://www.nbcnews.com/now/",
- ]
-
- should_not_match = [
- "https://www.nbcnews.com/",
- "http://www.nbcnews.com/",
- ]
| plugins.nbcnews: not live
### Checklist
- [X] This is a plugin issue and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest build from the master branch
### Description
Every time it start the same video but on the site it is live. Something was changed on the site few days ago.
### Debug log
```text
[cli][debug] OS: Linux-6.1.0-7-amd64-x86_64-with-glibc2.36
[cli][debug] Python: 3.11.2
[cli][debug] Streamlink: 5.3.1+84.g7978e4ab
[cli][debug] Dependencies:
[cli][debug] certifi: 2022.9.24
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.2
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.16.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.28.1
[cli][debug] urllib3: 1.26.12
[cli][debug] websocket-client: 1.2.3
[cli][debug] esprima: 4.0.1
[cli][debug] importlib-metadata: 4.12.0
[cli][debug] Arguments:
[cli][debug] url=https://www.nbcnews.com/now/
[cli][debug] --loglevel=debug
[cli][debug] --player=mpv
[cli][debug] --default-stream=['best']
[cli][info] Found matching plugin nbcnews for URL https://www.nbcnews.com/now/
[plugins.nbcnews][debug] API ID: 2007524
[utils.l10n][debug] Language code: ru_RU
[cli][info] Available streams: 144p_alt (worst), 144p, 216p_alt, 216p, 288p_alt, 288p, 360p_alt, 360p, 504p_alt, 504p, 576p_alt, 576p, 720p_alt, 720p, 1080p_alt, 1080p (best)
[cli][info] Opening stream: 1080p (hls)
[cli][info] Starting player: mpv
[stream.hls][debug] Reloading playlist
[cli][debug] Pre-buffering 8192 bytes
[stream.hls][debug] First Sequence: 1786423; Last Sequence: 1786722
[stream.hls][debug] Start offset: 0; Duration: None; Start Sequence: 1786423; End Sequence: 1786722
[stream.hls][debug] Adding segment 1786423 to queue
```
| It looks to me like they've switched to a new DRM based system with no clear options available.
Here's the API data:
<details><summary>API data</summary>
```
{
"NEWS_NOW": {
"serviceKey": "5676009166762707117",
"assetMetadata": {
"channelId": "NBC News Now",
"channelName": "nbc_news_now",
"programmeId": "",
"programmeTitle": "",
"duration": 0
},
"assets": {
"hlsCBCS": {
"drm": {
"resourceID": "not_required",
"encryptionData": "US-AFFILIATE-LINEAR-1-CBCS"
},
"transport": "HLS",
"encryptionType": "CBCS",
"container": "ISOBMFF",
"adCompatibility": {
"encodingProfile": "cmaf-hls-30fps-NBCS-Oneapp-v1"
},
"url": "https://live-oneapp-prd-news.akamaized.net/Content/CMAF_OL1-CBC-4s/Live/channel(nnn)/master.m3u8"
},
"hlsCENC": {
"drm": {
"resourceID": "not_required",
"encryptionData": "US-AFFILIATE-LINEAR-1-CENC"
},
"transport": "HLS",
"encryptionType": "CENC",
"container": "ISOBMFF",
"adCompatibility": {
"encodingProfile": "cmaf-hls-30fps-NBCS-Oneapp-v1"
},
"url": "https://live-oneapp-prd-news.akamaized.net/Content/CMAF_OL1-CTR-4s/Live/channel(nnn)/master.m3u8"
},
"dash": {
"drm": {
"resourceID": "not_required",
"encryptionData": "US-AFFILIATE-LINEAR-1-CENC"
},
"transport": "DASH",
"encryptionType": "CENC",
"container": "ISOBMFF",
"adCompatibility": {
"encodingProfile": "cmaf-dash-30fps-NBCS-Oneapp-v1"
},
"url": "https://live-oneapp-prd-news.akamaized.net/Content/CMAF_OL1-CTR-4s/Live/channel(nnn)/master.mpd"
}
}
},
"MSNBC_TVE": {
"serviceKey": "5608086763860174117",
"assetMetadata": {
"channelId": "MSNBC",
"channelName": "msnbc",
"programmeId": "",
"programmeTitle": "",
"duration": 0
},
"assets": {
"hlsCBCS": {
"drm": {
"resourceID": "not_required",
"encryptionData": "US-AFFILIATE-LINEAR-1-CBCS"
},
"transport": "HLS",
"encryptionType": "CBCS",
"container": "ISOBMFF",
"adCompatibility": {
"encodingProfile": "cmaf-hls-30fps-NBCS-Oneapp-v1"
},
"url": "https://live-oneapp-prd-news.akamaized.net/Content/CMAF_OL1-CBC-4s/Live/channel(msnbc)/master.m3u8"
},
"hlsCENC": {
"drm": {
"resourceID": "not_required",
"encryptionData": "US-AFFILIATE-LINEAR-1-CENC"
},
"transport": "HLS",
"encryptionType": "CENC",
"container": "ISOBMFF",
"adCompatibility": {
"encodingProfile": "cmaf-hls-30fps-NBCS-Oneapp-v1"
},
"url": "https://live-oneapp-prd-news.akamaized.net/Content/CMAF_OL1-CTR-4s/Live/channel(msnbc)/master.m3u8"
},
"dash": {
"drm": {
"resourceID": "not_required",
"encryptionData": "US-AFFILIATE-LINEAR-1-CENC"
},
"transport": "DASH",
"encryptionType": "CENC",
"container": "ISOBMFF",
"adCompatibility": {
"encodingProfile": "cmaf-dash-30fps-NBCS-Oneapp-v1"
},
"url": "https://live-oneapp-prd-news.akamaized.net/Content/CMAF_OL1-CTR-4s/Live/channel(msnbc)/master.mpd"
}
}
},
"TODAY_ALL_DAY": {
"serviceKey": "7989219137174638117",
"assetMetadata": {
"channelId": "Today",
"channelName": "today_all_day",
"programmeId": "",
"programmeTitle": "",
"duration": 0
},
"assets": {
"hlsCBCS": {
"drm": {
"resourceID": "not_required",
"encryptionData": "US-AFFILIATE-LINEAR-1-CBCS"
},
"transport": "HLS",
"encryptionType": "CBCS",
"container": "ISOBMFF",
"adCompatibility": {
"encodingProfile": "cmaf-hls-30fps-NBCS-Oneapp-v1"
},
"url": "https://live-oneapp-prd-news.akamaized.net/Content/CMAF_OL1-CBC-4s/Live/channel(todayallday)/master.m3u8"
},
"hlsCENC": {
"drm": {
"resourceID": "not_required",
"encryptionData": "US-AFFILIATE-LINEAR-1-CENC"
},
"transport": "HLS",
"encryptionType": "CENC",
"container": "ISOBMFF",
"adCompatibility": {
"encodingProfile": "cmaf-hls-30fps-NBCS-Oneapp-v1"
},
"url": "https://live-oneapp-prd-news.akamaized.net/Content/CMAF_OL1-CTR-4s/Live/channel(todayallday)/master.m3u8"
},
"dash": {
"drm": {
"resourceID": "not_required",
"encryptionData": "US-AFFILIATE-LINEAR-1-CENC"
},
"transport": "DASH",
"encryptionType": "CENC",
"container": "ISOBMFF",
"adCompatibility": {
"encodingProfile": "cmaf-dash-30fps-NBCS-Oneapp-v1"
},
"url": "https://live-oneapp-prd-news.akamaized.net/Content/CMAF_OL1-CTR-4s/Live/channel(todayallday)/master.mpd"
}
}
}
}
```
</details>
And these are the playlists/manifest for NNN:
<details><summary>hlsCBCS</summary>
```
#EXTM3U
#EXT-X-VERSION:4
#EXT-X-INDEPENDENT-SEGMENTS
#EXT-X-SESSION-KEY:METHOD=SAMPLE-AES,KEYFORMAT="com.apple.streamingkeydelivery",KEYFORMATVERSIONS="1",URI="skd://d3fef8ce2f593af0a29f6e4a522c6555",IV=0xd3fef8ce2f593af0a29f6e4a522c6555
#EXT-X-MEDIA:TYPE=AUDIO,GROUP-ID="audio1",NAME="English",DEFAULT=YES,AUTOSELECT=YES,LANGUAGE="en",URI="08_program.m3u8"
#EXT-X-MEDIA:TYPE=AUDIO,GROUP-ID="audio2",NAME="English",DEFAULT=YES,AUTOSELECT=YES,LANGUAGE="en",URI="07_program.m3u8"
#EXT-X-MEDIA:TYPE=CLOSED-CAPTIONS,GROUP-ID="cc",NAME="English",DEFAULT=NO,AUTOSELECT=YES,LANGUAGE="en",INSTREAM-ID="CC1"
#EXT-X-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=535200,CODECS="avc1.4d4015,mp4a.40.2",RESOLUTION=512x288,FRAME-RATE=29.970,AUDIO="audio1",AVERAGE-BANDWIDTH=446000,CLOSED-CAPTIONS="cc"
01_program.m3u8
#EXT-X-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=650400,CODECS="avc1.4d4015,ec-3",RESOLUTION=512x288,FRAME-RATE=29.970,AUDIO="audio2",AVERAGE-BANDWIDTH=542000,CLOSED-CAPTIONS="cc"
01_program.m3u8
#EXT-X-I-FRAME-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=40650,CODECS="avc1.4d4015",RESOLUTION=512x288,AVERAGE-BANDWIDTH=33875,URI="01-iframe_program.m3u8"
#EXT-X-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=1147200,CODECS="avc1.4d401e,mp4a.40.2",RESOLUTION=768x432,FRAME-RATE=29.970,AUDIO="audio1",AVERAGE-BANDWIDTH=956000,CLOSED-CAPTIONS="cc"
02_program.m3u8
#EXT-X-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=1262400,CODECS="avc1.4d401e,ec-3",RESOLUTION=768x432,FRAME-RATE=29.970,AUDIO="audio2",AVERAGE-BANDWIDTH=1052000,CLOSED-CAPTIONS="cc"
02_program.m3u8
#EXT-X-I-FRAME-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=78900,CODECS="avc1.4d401e",RESOLUTION=768x432,AVERAGE-BANDWIDTH=65750,URI="02-iframe_program.m3u8"
#EXT-X-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=2335200,CODECS="avc1.4d401f,mp4a.40.2",RESOLUTION=960x540,FRAME-RATE=29.970,AUDIO="audio1",AVERAGE-BANDWIDTH=1946000,CLOSED-CAPTIONS="cc"
03_program.m3u8
#EXT-X-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=2450400,CODECS="avc1.4d401f,ec-3",RESOLUTION=960x540,FRAME-RATE=29.970,AUDIO="audio2",AVERAGE-BANDWIDTH=2042000,CLOSED-CAPTIONS="cc"
03_program.m3u8
#EXT-X-I-FRAME-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=153150,CODECS="avc1.4d401f",RESOLUTION=960x540,AVERAGE-BANDWIDTH=127625,URI="03-iframe_program.m3u8"
#EXT-X-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=3715200,CODECS="avc1.64001f,mp4a.40.2",RESOLUTION=960x540,FRAME-RATE=29.970,AUDIO="audio1",AVERAGE-BANDWIDTH=3096000,CLOSED-CAPTIONS="cc"
04_program.m3u8
#EXT-X-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=3830400,CODECS="avc1.64001f,ec-3",RESOLUTION=960x540,FRAME-RATE=29.970,AUDIO="audio2",AVERAGE-BANDWIDTH=3192000,CLOSED-CAPTIONS="cc"
04_program.m3u8
#EXT-X-I-FRAME-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=239400,CODECS="avc1.64001f",RESOLUTION=960x540,AVERAGE-BANDWIDTH=199500,URI="04-iframe_program.m3u8"
#EXT-X-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=5911200,CODECS="avc1.64001f,mp4a.40.2",RESOLUTION=1280x720,FRAME-RATE=29.970,AUDIO="audio1",AVERAGE-BANDWIDTH=4926000,CLOSED-CAPTIONS="cc"
05_program.m3u8
#EXT-X-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=6026400,CODECS="avc1.64001f,ec-3",RESOLUTION=1280x720,FRAME-RATE=29.970,AUDIO="audio2",AVERAGE-BANDWIDTH=5022000,CLOSED-CAPTIONS="cc"
05_program.m3u8
#EXT-X-I-FRAME-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=376650,CODECS="avc1.64001f",RESOLUTION=1280x720,AVERAGE-BANDWIDTH=313875,URI="05-iframe_program.m3u8"
#EXT-X-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=9511200,CODECS="avc1.640028,mp4a.40.2",RESOLUTION=1920x1080,FRAME-RATE=29.970,AUDIO="audio1",AVERAGE-BANDWIDTH=7926000,CLOSED-CAPTIONS="cc"
06_program.m3u8
#EXT-X-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=9626400,CODECS="avc1.640028,ec-3",RESOLUTION=1920x1080,FRAME-RATE=29.970,AUDIO="audio2",AVERAGE-BANDWIDTH=8022000,CLOSED-CAPTIONS="cc"
06_program.m3u8
#EXT-X-I-FRAME-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=601650,CODECS="avc1.640028",RESOLUTION=1920x1080,AVERAGE-BANDWIDTH=501375,URI="06-iframe_program.m3u8"
```
</details>
<details><summary>hlsCENC</summary>
```
#EXTM3U
#EXT-X-VERSION:4
#EXT-X-INDEPENDENT-SEGMENTS
#EXT-X-SESSION-KEY:METHOD=SAMPLE-AES-CTR,KEYFORMAT="urn:uuid:edef8ba9-79d6-4ace-a3c8-27dcd51d21ed",KEYFORMATVERSIONS="1",URI="data:text/plain;base64,AAAAV3Bzc2gAAAAA7e+LqXnWSs6jyCfc1R0h7QAAADcIARIQ0/74zi9ZOvCin25KUixlVRoLYnV5ZHJta2V5b3MiENP++M4vWTrwop9uSlIsZVUqAkhE"
#EXT-X-SESSION-KEY:METHOD=SAMPLE-AES,KEYFORMAT="com.microsoft.playready",KEYFORMATVERSIONS="1",URI="data:text/plain;charset=UTF-16;base64,AAAD4nBzc2gAAAAAmgTweZhAQoarkuZb4IhflQAAA8LCAwAAAQABALgDPABXAFIATQBIAEUAQQBEAEUAUgAgAHgAbQBsAG4AcwA9ACIAaAB0AHQAcAA6AC8ALwBzAGMAaABlAG0AYQBzAC4AbQBpAGMAcgBvAHMAbwBmAHQALgBjAG8AbQAvAEQAUgBNAC8AMgAwADAANwAvADAAMwAvAFAAbABhAHkAUgBlAGEAZAB5AEgAZQBhAGQAZQByACIAIAB2AGUAcgBzAGkAbwBuAD0AIgA0AC4AMAAuADAALgAwACIAPgA8AEQAQQBUAEEAPgA8AFAAUgBPAFQARQBDAFQASQBOAEYATwA+ADwASwBFAFkATABFAE4APgAxADYAPAAvAEsARQBZAEwARQBOAD4APABBAEwARwBJAEQAPgBBAEUAUwBDAFQAUgA8AC8AQQBMAEcASQBEAD4APAAvAFAAUgBPAFQARQBDAFQASQBOAEYATwA+ADwASwBJAEQAPgB6AHYAagArADAAMQBrAHYAOABEAHEAaQBuADIANQBLAFUAaQB4AGwAVgBRAD0APQA8AC8ASwBJAEQAPgA8AEwAQQBfAFUAUgBMAD4AaAB0AHQAcAA6AC8ALwBwAHIALQBrAGUAeQBvAHMALgBsAGkAYwBlAG4AcwBlAGsAZQB5AHMAZQByAHYAZQByAC4AYwBvAG0ALwBjAG8AcgBlAC8AcgBpAGcAaAB0AHMAbQBhAG4AYQBnAGUAcgAuAGEAcwBtAHgAPAAvAEwAQQBfAFUAUgBMAD4APABEAFMAXwBJAEQAPgBWAGwAUgA3AEkAZABzAEkASgBFAHUAUgBkADAANgBMAGEAcQBzADIAagB3AD0APQA8AC8ARABTAF8ASQBEAD4APABDAFUAUwBUAE8ATQBBAFQAVABSAEkAQgBVAFQARQBTACAAeABtAGwAbgBzAD0AIgAiAD4APABDAEkARAA+AHoAdgBqACsAMAAxAGsAdgA4AEQAcQBpAG4AMgA1AEsAVQBpAHgAbABWAFEAPQA9ADwALwBDAEkARAA+ADwARABSAE0AVABZAFAARQA+AHMAbQBvAG8AdABoADwALwBEAFIATQBUAFkAUABFAD4APAAvAEMAVQBTAFQATwBNAEEAVABUAFIASQBCAFUAVABFAFMAPgA8AEMASABFAEMASwBTAFUATQA+AHEAdwA3AHgAOABMAEMAYQBVAE0ATQA9ADwALwBDAEgARQBDAEsAUwBVAE0APgA8AC8ARABBAFQAQQA+ADwALwBXAFIATQBIAEUAQQBEAEUAUgA+AA=="
#EXT-X-MEDIA:TYPE=AUDIO,GROUP-ID="audio1",NAME="English",DEFAULT=YES,AUTOSELECT=YES,LANGUAGE="en",URI="08_program.m3u8"
#EXT-X-MEDIA:TYPE=AUDIO,GROUP-ID="audio2",NAME="English",DEFAULT=YES,AUTOSELECT=YES,LANGUAGE="en",URI="07_program.m3u8"
#EXT-X-MEDIA:TYPE=CLOSED-CAPTIONS,GROUP-ID="cc",NAME="English",DEFAULT=NO,AUTOSELECT=YES,LANGUAGE="en",INSTREAM-ID="CC1"
#EXT-X-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=535200,CODECS="avc1.4d4015,mp4a.40.2",RESOLUTION=512x288,FRAME-RATE=29.970,AUDIO="audio1",AVERAGE-BANDWIDTH=446000,CLOSED-CAPTIONS="cc"
01_program.m3u8
#EXT-X-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=650400,CODECS="avc1.4d4015,ec-3",RESOLUTION=512x288,FRAME-RATE=29.970,AUDIO="audio2",AVERAGE-BANDWIDTH=542000,CLOSED-CAPTIONS="cc"
01_program.m3u8
#EXT-X-I-FRAME-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=40650,CODECS="avc1.4d4015",RESOLUTION=512x288,AVERAGE-BANDWIDTH=33875,URI="01-iframe_program.m3u8"
#EXT-X-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=1147200,CODECS="avc1.4d401e,mp4a.40.2",RESOLUTION=768x432,FRAME-RATE=29.970,AUDIO="audio1",AVERAGE-BANDWIDTH=956000,CLOSED-CAPTIONS="cc"
02_program.m3u8
#EXT-X-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=1262400,CODECS="avc1.4d401e,ec-3",RESOLUTION=768x432,FRAME-RATE=29.970,AUDIO="audio2",AVERAGE-BANDWIDTH=1052000,CLOSED-CAPTIONS="cc"
02_program.m3u8
#EXT-X-I-FRAME-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=78900,CODECS="avc1.4d401e",RESOLUTION=768x432,AVERAGE-BANDWIDTH=65750,URI="02-iframe_program.m3u8"
#EXT-X-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=2335200,CODECS="avc1.4d401f,mp4a.40.2",RESOLUTION=960x540,FRAME-RATE=29.970,AUDIO="audio1",AVERAGE-BANDWIDTH=1946000,CLOSED-CAPTIONS="cc"
03_program.m3u8
#EXT-X-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=2450400,CODECS="avc1.4d401f,ec-3",RESOLUTION=960x540,FRAME-RATE=29.970,AUDIO="audio2",AVERAGE-BANDWIDTH=2042000,CLOSED-CAPTIONS="cc"
03_program.m3u8
#EXT-X-I-FRAME-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=153150,CODECS="avc1.4d401f",RESOLUTION=960x540,AVERAGE-BANDWIDTH=127625,URI="03-iframe_program.m3u8"
#EXT-X-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=3715200,CODECS="avc1.64001f,mp4a.40.2",RESOLUTION=960x540,FRAME-RATE=29.970,AUDIO="audio1",AVERAGE-BANDWIDTH=3096000,CLOSED-CAPTIONS="cc"
04_program.m3u8
#EXT-X-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=3830400,CODECS="avc1.64001f,ec-3",RESOLUTION=960x540,FRAME-RATE=29.970,AUDIO="audio2",AVERAGE-BANDWIDTH=3192000,CLOSED-CAPTIONS="cc"
04_program.m3u8
#EXT-X-I-FRAME-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=239400,CODECS="avc1.64001f",RESOLUTION=960x540,AVERAGE-BANDWIDTH=199500,URI="04-iframe_program.m3u8"
#EXT-X-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=5911200,CODECS="avc1.64001f,mp4a.40.2",RESOLUTION=1280x720,FRAME-RATE=29.970,AUDIO="audio1",AVERAGE-BANDWIDTH=4926000,CLOSED-CAPTIONS="cc"
05_program.m3u8
#EXT-X-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=6026400,CODECS="avc1.64001f,ec-3",RESOLUTION=1280x720,FRAME-RATE=29.970,AUDIO="audio2",AVERAGE-BANDWIDTH=5022000,CLOSED-CAPTIONS="cc"
05_program.m3u8
#EXT-X-I-FRAME-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=376650,CODECS="avc1.64001f",RESOLUTION=1280x720,AVERAGE-BANDWIDTH=313875,URI="05-iframe_program.m3u8"
#EXT-X-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=9511200,CODECS="avc1.640028,mp4a.40.2",RESOLUTION=1920x1080,FRAME-RATE=29.970,AUDIO="audio1",AVERAGE-BANDWIDTH=7926000,CLOSED-CAPTIONS="cc"
06_program.m3u8
#EXT-X-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=9626400,CODECS="avc1.640028,ec-3",RESOLUTION=1920x1080,FRAME-RATE=29.970,AUDIO="audio2",AVERAGE-BANDWIDTH=8022000,CLOSED-CAPTIONS="cc"
06_program.m3u8
#EXT-X-I-FRAME-STREAM-INF:PROGRAM-ID=1,BANDWIDTH=601650,CODECS="avc1.640028",RESOLUTION=1920x1080,AVERAGE-BANDWIDTH=501375,URI="06-iframe_program.m3u8"
```
</details>
<details><summary>dash</summary>
```
<?xml version="1.0" encoding="UTF-8"?>
<MPD profiles="urn:mpeg:dash:profile:isoff-live:2011" type="dynamic" availabilityStartTime="2022-06-24T21:25:44Z" minimumUpdatePeriod="PT3.904S" minBufferTime="PT7.808S" publishTime="2023-04-07T19:08:35.568Z" timeShiftBufferDepth="PT2M" xmlns="urn:mpeg:dash:schema:mpd:2011" xmlns:cenc="urn:mpeg:cenc:2013" xmlns:mspr="urn:microsoft:playready" xmlns:scte35="urn:scte:scte35:2014:xml+bin" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="urn:mpeg:dash:schema:mpd:2011 DASH-MPD.xsd" xmlns:dolby="http://www.dolby.com/ns/online/DASH">
<Period start="PT6885H34M38.6221576S" id="55962">
<EventStream timescale="10000000" schemeIdUri="urn:scte:scte35:2014:xml+bin">
<Event id="5">
<scte35:Signal>
<scte35:Binary>/DBCAAAAAAAAAAAABQb+7cq7RAAsAipDVUVJAAAABX//AADN+HIOFm5ld3Nub3dfRVAwNDY4OTMzNTAwMjMxBgCql9/O</scte35:Binary>
</scte35:Signal>
</Event>
<Event id="1">
<scte35:Signal>
<scte35:Binary>/DBCAAAAAAAAAP/wBQb+7cq7RAAsAipDVUVJAAAAAX//AADN+HIOFm5ld3Nub3dfRVAwNDY4OTMzNTAwMjMjBgDWWnKh</scte35:Binary>
</scte35:Signal>
</Event>
</EventStream>
<AdaptationSet mimeType="video/mp4" startWithSAP="1" segmentAlignment="true" par="16:9">
<ContentProtection schemeIdUri="urn:mpeg:dash:mp4protection:2011" value="cenc" cenc:default_KID="d3fef8ce-2f59-3af0-a29f-6e4a522c6555"></ContentProtection>
<ContentProtection schemeIdUri="urn:uuid:edef8ba9-79d6-4ace-a3c8-27dcd51d21ed"><cenc:pssh xmlns:cenc="urn:mpeg:cenc:2013">AAAAV3Bzc2gAAAAA7e+LqXnWSs6jyCfc1R0h7QAAADcIARIQ0/74zi9ZOvCin25KUixlVRoLYnV5ZHJta2V5b3MiENP++M4vWTrwop9uSlIsZVUqAkhE</cenc:pssh></ContentProtection>
<ContentProtection schemeIdUri="urn:uuid:9a04f079-9840-4286-ab92-e65be0885f95"><cenc:pssh xmlns:cenc="urn:mpeg:cenc:2013">AAAD4nBzc2gAAAAAmgTweZhAQoarkuZb4IhflQAAA8LCAwAAAQABALgDPABXAFIATQBIAEUAQQBEAEUAUgAgAHgAbQBsAG4AcwA9ACIAaAB0AHQAcAA6AC8ALwBzAGMAaABlAG0AYQBzAC4AbQBpAGMAcgBvAHMAbwBmAHQALgBjAG8AbQAvAEQAUgBNAC8AMgAwADAANwAvADAAMwAvAFAAbABhAHkAUgBlAGEAZAB5AEgAZQBhAGQAZQByACIAIAB2AGUAcgBzAGkAbwBuAD0AIgA0AC4AMAAuADAALgAwACIAPgA8AEQAQQBUAEEAPgA8AFAAUgBPAFQARQBDAFQASQBOAEYATwA+ADwASwBFAFkATABFAE4APgAxADYAPAAvAEsARQBZAEwARQBOAD4APABBAEwARwBJAEQAPgBBAEUAUwBDAFQAUgA8AC8AQQBMAEcASQBEAD4APAAvAFAAUgBPAFQARQBDAFQASQBOAEYATwA+ADwASwBJAEQAPgB6AHYAagArADAAMQBrAHYAOABEAHEAaQBuADIANQBLAFUAaQB4AGwAVgBRAD0APQA8AC8ASwBJAEQAPgA8AEwAQQBfAFUAUgBMAD4AaAB0AHQAcAA6AC8ALwBwAHIALQBrAGUAeQBvAHMALgBsAGkAYwBlAG4AcwBlAGsAZQB5AHMAZQByAHYAZQByAC4AYwBvAG0ALwBjAG8AcgBlAC8AcgBpAGcAaAB0AHMAbQBhAG4AYQBnAGUAcgAuAGEAcwBtAHgAPAAvAEwAQQBfAFUAUgBMAD4APABEAFMAXwBJAEQAPgBWAGwAUgA3AEkAZABzAEkASgBFAHUAUgBkADAANgBMAGEAcQBzADIAagB3AD0APQA8AC8ARABTAF8ASQBEAD4APABDAFUAUwBUAE8ATQBBAFQAVABSAEkAQgBVAFQARQBTACAAeABtAGwAbgBzAD0AIgAiAD4APABDAEkARAA+AHoAdgBqACsAMAAxAGsAdgA4AEQAcQBpAG4AMgA1AEsAVQBpAHgAbABWAFEAPQA9ADwALwBDAEkARAA+ADwARABSAE0AVABZAFAARQA+AHMAbQBvAG8AdABoADwALwBEAFIATQBUAFkAUABFAD4APAAvAEMAVQBTAFQATwBNAEEAVABUAFIASQBCAFUAVABFAFMAPgA8AEMASABFAEMASwBTAFUATQA+AHEAdwA3AHgAOABMAEMAYQBVAE0ATQA9ADwALwBDAEgARQBDAEsAUwBVAE0APgA8AC8ARABBAFQAQQA+ADwALwBXAFIATQBIAEUAQQBEAEUAUgA+AA==</cenc:pssh></ContentProtection>
<InbandEventStream schemeIdUri="urn:scte:scte35:2013:xml" value="1"></InbandEventStream>
<Accessibility schemeIdUri="urn:scte:dash:cc:cea-608:2015" value="CC1=ENG"></Accessibility>
<SegmentTemplate timescale="10000000" presentationTimeOffset="247880786221576" media="$RepresentationID$_Segment-$Number$.mp4" initialization="$RepresentationID$_init.m4i" startNumber="6351092">
<SegmentTimeline>
<S t="247884614045576" d="39039000" r="30"></S>
</SegmentTimeline>
</SegmentTemplate>
<Representation width="512" height="288" frameRate="30000/1001" codecs="avc1.4d4015" scanType="progressive" sar="1:1" id="1656105933133item-01item" bandwidth="350000"></Representation>
<Representation width="768" height="432" frameRate="30000/1001" codecs="avc1.4d401e" scanType="progressive" sar="1:1" id="1656105933133item-02item" bandwidth="860000"></Representation>
<Representation width="960" height="540" frameRate="30000/1001" codecs="avc1.4d401f" scanType="progressive" sar="1:1" id="1656105933133item-03item" bandwidth="1850000"></Representation>
<Representation width="960" height="540" frameRate="30000/1001" codecs="avc1.64001f" scanType="progressive" sar="1:1" id="1656105933133item-04item" bandwidth="3000000"></Representation>
<Representation width="1280" height="720" frameRate="30000/1001" codecs="avc1.64001f" scanType="progressive" sar="1:1" id="1656105933133item-05item" bandwidth="4830000"></Representation>
<Representation width="1920" height="1080" frameRate="30000/1001" codecs="avc1.640028" scanType="progressive" sar="1:1" id="1656105933133item-06item" bandwidth="7830000"></Representation>
</AdaptationSet>
<AdaptationSet mimeType="audio/mp4" startWithSAP="1" lang="eng" segmentAlignment="true">
<ContentProtection schemeIdUri="urn:mpeg:dash:mp4protection:2011" value="cenc" cenc:default_KID="d3fef8ce-2f59-3af0-a29f-6e4a522c6555"></ContentProtection>
<ContentProtection schemeIdUri="urn:uuid:edef8ba9-79d6-4ace-a3c8-27dcd51d21ed"><cenc:pssh xmlns:cenc="urn:mpeg:cenc:2013">AAAAV3Bzc2gAAAAA7e+LqXnWSs6jyCfc1R0h7QAAADcIARIQ0/74zi9ZOvCin25KUixlVRoLYnV5ZHJta2V5b3MiENP++M4vWTrwop9uSlIsZVUqAkhE</cenc:pssh></ContentProtection>
<ContentProtection schemeIdUri="urn:uuid:9a04f079-9840-4286-ab92-e65be0885f95"><cenc:pssh xmlns:cenc="urn:mpeg:cenc:2013">AAAD4nBzc2gAAAAAmgTweZhAQoarkuZb4IhflQAAA8LCAwAAAQABALgDPABXAFIATQBIAEUAQQBEAEUAUgAgAHgAbQBsAG4AcwA9ACIAaAB0AHQAcAA6AC8ALwBzAGMAaABlAG0AYQBzAC4AbQBpAGMAcgBvAHMAbwBmAHQALgBjAG8AbQAvAEQAUgBNAC8AMgAwADAANwAvADAAMwAvAFAAbABhAHkAUgBlAGEAZAB5AEgAZQBhAGQAZQByACIAIAB2AGUAcgBzAGkAbwBuAD0AIgA0AC4AMAAuADAALgAwACIAPgA8AEQAQQBUAEEAPgA8AFAAUgBPAFQARQBDAFQASQBOAEYATwA+ADwASwBFAFkATABFAE4APgAxADYAPAAvAEsARQBZAEwARQBOAD4APABBAEwARwBJAEQAPgBBAEUAUwBDAFQAUgA8AC8AQQBMAEcASQBEAD4APAAvAFAAUgBPAFQARQBDAFQASQBOAEYATwA+ADwASwBJAEQAPgB6AHYAagArADAAMQBrAHYAOABEAHEAaQBuADIANQBLAFUAaQB4AGwAVgBRAD0APQA8AC8ASwBJAEQAPgA8AEwAQQBfAFUAUgBMAD4AaAB0AHQAcAA6AC8ALwBwAHIALQBrAGUAeQBvAHMALgBsAGkAYwBlAG4AcwBlAGsAZQB5AHMAZQByAHYAZQByAC4AYwBvAG0ALwBjAG8AcgBlAC8AcgBpAGcAaAB0AHMAbQBhAG4AYQBnAGUAcgAuAGEAcwBtAHgAPAAvAEwAQQBfAFUAUgBMAD4APABEAFMAXwBJAEQAPgBWAGwAUgA3AEkAZABzAEkASgBFAHUAUgBkADAANgBMAGEAcQBzADIAagB3AD0APQA8AC8ARABTAF8ASQBEAD4APABDAFUAUwBUAE8ATQBBAFQAVABSAEkAQgBVAFQARQBTACAAeABtAGwAbgBzAD0AIgAiAD4APABDAEkARAA+AHoAdgBqACsAMAAxAGsAdgA4AEQAcQBpAG4AMgA1AEsAVQBpAHgAbABWAFEAPQA9ADwALwBDAEkARAA+ADwARABSAE0AVABZAFAARQA+AHMAbQBvAG8AdABoADwALwBEAFIATQBUAFkAUABFAD4APAAvAEMAVQBTAFQATwBNAEEAVABUAFIASQBCAFUAVABFAFMAPgA8AEMASABFAEMASwBTAFUATQA+AHEAdwA3AHgAOABMAEMAYQBVAE0ATQA9ADwALwBDAEgARQBDAEsAUwBVAE0APgA8AC8ARABBAFQAQQA+ADwALwBXAFIATQBIAEUAQQBEAEUAUgA+AA==</cenc:pssh></ContentProtection>
<InbandEventStream schemeIdUri="https://aomedia.org/emsg/ID3" value="www.nielsen.com:id3:v1"></InbandEventStream>
<SegmentTemplate timescale="10000000" presentationTimeOffset="247880786427296" media="$RepresentationID$_Segment-$Number$.mp4" initialization="$RepresentationID$_init.m4i" startNumber="6351092">
<SegmentTimeline>
<S t="247884614267296" d="39040000" r="30"></S>
</SegmentTimeline>
</SegmentTemplate>
<Representation audioSamplingRate="48000" codecs="ec-3" id="1656105933133item-07item" bandwidth="192000">
<AudioChannelConfiguration schemeIdUri="tag:dolby.com,2014:dash:audio_channel_configuration:2011" value="F801" />
</Representation>
</AdaptationSet>
<AdaptationSet mimeType="audio/mp4" startWithSAP="1" lang="eng" segmentAlignment="true">
<ContentProtection schemeIdUri="urn:mpeg:dash:mp4protection:2011" value="cenc" cenc:default_KID="d3fef8ce-2f59-3af0-a29f-6e4a522c6555"></ContentProtection>
<ContentProtection schemeIdUri="urn:uuid:edef8ba9-79d6-4ace-a3c8-27dcd51d21ed"><cenc:pssh xmlns:cenc="urn:mpeg:cenc:2013">AAAAV3Bzc2gAAAAA7e+LqXnWSs6jyCfc1R0h7QAAADcIARIQ0/74zi9ZOvCin25KUixlVRoLYnV5ZHJta2V5b3MiENP++M4vWTrwop9uSlIsZVUqAkhE</cenc:pssh></ContentProtection>
<ContentProtection schemeIdUri="urn:uuid:9a04f079-9840-4286-ab92-e65be0885f95"><cenc:pssh xmlns:cenc="urn:mpeg:cenc:2013">AAAD4nBzc2gAAAAAmgTweZhAQoarkuZb4IhflQAAA8LCAwAAAQABALgDPABXAFIATQBIAEUAQQBEAEUAUgAgAHgAbQBsAG4AcwA9ACIAaAB0AHQAcAA6AC8ALwBzAGMAaABlAG0AYQBzAC4AbQBpAGMAcgBvAHMAbwBmAHQALgBjAG8AbQAvAEQAUgBNAC8AMgAwADAANwAvADAAMwAvAFAAbABhAHkAUgBlAGEAZAB5AEgAZQBhAGQAZQByACIAIAB2AGUAcgBzAGkAbwBuAD0AIgA0AC4AMAAuADAALgAwACIAPgA8AEQAQQBUAEEAPgA8AFAAUgBPAFQARQBDAFQASQBOAEYATwA+ADwASwBFAFkATABFAE4APgAxADYAPAAvAEsARQBZAEwARQBOAD4APABBAEwARwBJAEQAPgBBAEUAUwBDAFQAUgA8AC8AQQBMAEcASQBEAD4APAAvAFAAUgBPAFQARQBDAFQASQBOAEYATwA+ADwASwBJAEQAPgB6AHYAagArADAAMQBrAHYAOABEAHEAaQBuADIANQBLAFUAaQB4AGwAVgBRAD0APQA8AC8ASwBJAEQAPgA8AEwAQQBfAFUAUgBMAD4AaAB0AHQAcAA6AC8ALwBwAHIALQBrAGUAeQBvAHMALgBsAGkAYwBlAG4AcwBlAGsAZQB5AHMAZQByAHYAZQByAC4AYwBvAG0ALwBjAG8AcgBlAC8AcgBpAGcAaAB0AHMAbQBhAG4AYQBnAGUAcgAuAGEAcwBtAHgAPAAvAEwAQQBfAFUAUgBMAD4APABEAFMAXwBJAEQAPgBWAGwAUgA3AEkAZABzAEkASgBFAHUAUgBkADAANgBMAGEAcQBzADIAagB3AD0APQA8AC8ARABTAF8ASQBEAD4APABDAFUAUwBUAE8ATQBBAFQAVABSAEkAQgBVAFQARQBTACAAeABtAGwAbgBzAD0AIgAiAD4APABDAEkARAA+AHoAdgBqACsAMAAxAGsAdgA4AEQAcQBpAG4AMgA1AEsAVQBpAHgAbABWAFEAPQA9ADwALwBDAEkARAA+ADwARABSAE0AVABZAFAARQA+AHMAbQBvAG8AdABoADwALwBEAFIATQBUAFkAUABFAD4APAAvAEMAVQBTAFQATwBNAEEAVABUAFIASQBCAFUAVABFAFMAPgA8AEMASABFAEMASwBTAFUATQA+AHEAdwA3AHgAOABMAEMAYQBVAE0ATQA9ADwALwBDAEgARQBDAEsAUwBVAE0APgA8AC8ARABBAFQAQQA+ADwALwBXAFIATQBIAEUAQQBEAEUAUgA+AA==</cenc:pssh></ContentProtection>
<InbandEventStream schemeIdUri="https://aomedia.org/emsg/ID3" value="www.nielsen.com:id3:v1"></InbandEventStream>
<SegmentTemplate timescale="10000000" presentationTimeOffset="247880786427296" media="$RepresentationID$_Segment-$Number$.mp4" initialization="$RepresentationID$_init.m4i" startNumber="6351092">
<SegmentTimeline>
<S t="247884614053963" d="39040000" r="30"></S>
</SegmentTimeline>
</SegmentTemplate>
<Representation audioSamplingRate="48000" codecs="mp4a.40.2" id="1656105933133item-08item" bandwidth="96000">
<AudioChannelConfiguration schemeIdUri="urn:mpeg:dash:23003:3:audio_channel_configuration:2011" value="2" />
</Representation>
</AdaptationSet>
</Period>
<UTCTiming schemeIdUri="urn:mpeg:dash:utc:http-iso:2014" value="https://time.akamai.com/?iso&ms"></UTCTiming>
</MPD>
```
</details>
I'll submit a plugin removal PR later.
| 2023-04-08T09:55:03 |
streamlink/streamlink | 5,299 | streamlink__streamlink-5299 | [
"5297"
] | 5846abd6ec021eb0fc72250550c28809f79b9ac8 | diff --git a/src/streamlink/plugins/dogan.py b/src/streamlink/plugins/dogan.py
--- a/src/streamlink/plugins/dogan.py
+++ b/src/streamlink/plugins/dogan.py
@@ -33,34 +33,41 @@ class Dogan(Plugin):
]
API_URL_OLD = "/actions/media?id={id}"
- def _get_content_id(self):
- return self.session.http.get(
- self.url,
- schema=validate.Schema(
- validate.parse_html(),
- validate.any(
- validate.all(
- validate.xml_xpath_string("""
- .//div[@data-id][
- @data-live
- or @id='video-element'
- or @id='player-container'
- or contains(@class, 'player-container')
- ][1]/@data-id
- """),
- str,
- ),
- # xpath query needs to have a lower priority
- validate.all(
- validate.xml_xpath_string(
- ".//body[@data-content-id][1]/@data-content-id",
- ),
- str,
+ @staticmethod
+ def _get_hls_url(root):
+ schema = validate.Schema(
+ validate.xml_xpath_string(".//*[@data-live][contains(@data-url,'.m3u8')]/@data-url"),
+ )
+
+ return schema.validate(root)
+
+ @staticmethod
+ def _get_content_id(root):
+ schema=validate.Schema(
+ validate.any(
+ validate.all(
+ validate.xml_xpath_string("""
+ .//div[@data-id][
+ @data-live
+ or @id='video-element'
+ or @id='player-container'
+ or contains(@class, 'player-container')
+ ][1]/@data-id
+ """),
+ str,
+ ),
+ # xpath query needs to have a lower priority
+ validate.all(
+ validate.xml_xpath_string(
+ ".//body[@data-content-id][1]/@data-content-id",
),
+ str,
),
),
)
+ return schema.validate(root)
+
def _api_query_new(self, content_id, api_url):
url = urljoin(self.url, api_url.format(id=content_id))
data = self.session.http.get(
@@ -131,7 +138,7 @@ def _api_query_old(self, content_id):
return urljoin(service_url or default_service_url, secure_path)
- def _get_hls_url(self, content_id):
+ def _query_hls_url(self, content_id):
for idx, match in enumerate(self.matches[:len(self.API_URLS)]):
if match:
return self._api_query_new(content_id, self.API_URLS[idx])
@@ -139,18 +146,21 @@ def _get_hls_url(self, content_id):
return self._api_query_old(content_id)
def _get_streams(self):
- try:
- content_id = self._get_content_id()
- except PluginError:
- log.error("Could not find the content ID for this stream")
- return
+ root = self.session.http.get(self.url, schema=validate.Schema(validate.parse_html()))
- log.debug(f"Loading content: {content_id}")
- hls_url = self._get_hls_url(content_id)
+ hls_url = self._get_hls_url(root)
if not hls_url:
- return
+ try:
+ content_id = self._get_content_id(root)
+ except PluginError:
+ log.error("Could not find the content ID for this stream")
+ return
+
+ log.debug(f"Loading content: {content_id}")
+ hls_url = self._query_hls_url(content_id)
- return HLSStream.parse_variant_playlist(self.session, hls_url)
+ if hls_url:
+ return HLSStream.parse_variant_playlist(self.session, hls_url)
__plugin__ = Dogan
| plugins.dogan: CnnTurk and KanalD doesn't show live TV
### Checklist
- [X] This is a plugin issue and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest stable release
### Description
stulluk ~ $ streamlink --version
streamlink 5.4.0
stulluk ~ $
Thank you very much for this plugin. it works beautifully with "teve2" as follows:
`streamlink https://www.teve2.com.tr/canli-yayin best`
And it shows the live TV show.
However, it doesn't show liveTV streams of CnnTurk and KanalD.
To reproduce, one can compare played streams by checking live TV from the corresponding website via web browser.
I provide debug log below, but I am not sure if this is helpful at all, because below debug log is just fine, however played video is not live TV (some other advertisement is being played)
### Debug log
```text
stulluk ~ $ streamlink https://www.kanald.com.tr/canli-yayin best -l all
[13:14:12.746759][cli][debug] OS: Linux-6.2.10-060210-generic-x86_64-with-glibc2.35
[13:14:12.746857][cli][debug] Python: 3.10.6
[13:14:12.746898][cli][debug] Streamlink: 5.4.0
[13:14:12.747355][cli][debug] Dependencies:
[13:14:12.749140][cli][debug] certifi: 2020.6.20
[13:14:12.749491][cli][debug] isodate: 0.6.1
[13:14:12.749835][cli][debug] lxml: 4.8.0
[13:14:12.750241][cli][debug] pycountry: 20.7.3
[13:14:12.750469][cli][debug] pycryptodome: 3.17
[13:14:12.751129][cli][debug] PySocks: 1.7.1
[13:14:12.751392][cli][debug] requests: 2.28.2
[13:14:12.753761][cli][debug] urllib3: 1.26.5
[13:14:12.754057][cli][debug] websocket-client: 1.2.3
[13:14:12.754299][cli][debug] importlib-metadata: 4.6.4
[13:14:12.754439][cli][debug] Arguments:
[13:14:12.754480][cli][debug] url=https://www.kanald.com.tr/canli-yayin
[13:14:12.754517][cli][debug] stream=['best']
[13:14:12.754558][cli][debug] --loglevel=all
[13:14:12.754722][cli][info] Found matching plugin dogan for URL https://www.kanald.com.tr/canli-yayin
[13:14:12.864279][plugins.dogan][debug] Loading content: 542410a361361f36f4c3fcf1
[13:14:12.951559][utils.l10n][debug] Language code: en_US
[13:14:13.319823][stream.hls_playlist][all] #EXT-X-VERSION:3
[13:14:13.319996][stream.hls_playlist][all] #EXT-X-STREAM-INF:BANDWIDTH=510400,RESOLUTION=640x360,CODECS="avc1.42c01e,mp4a.40.2"
[13:14:13.320122][stream.hls_playlist][all] kanald-v0/playlist.m3u8?live=true&app=com.kanald&min=0&max=1250
[13:14:13.320311][stream.hls_playlist][all] #EXT-X-STREAM-INF:BANDWIDTH=1445400,RESOLUTION=1280x720,CODECS="avc1.4d401f,mp4a.40.2"
[13:14:13.320415][stream.hls_playlist][all] kanald-v1/playlist.m3u8?live=true&app=com.kanald&min=0&max=1250
[13:14:13.321506][cli][info] Available streams: 360p (worst), 720p (best)
[13:14:13.321740][cli][info] Opening stream: 720p (hls)
[13:14:13.321884][cli][info] Starting player: /usr/bin/vlc
[13:14:13.322923][stream.hls][debug] Reloading playlist
[13:14:13.323106][cli][debug] Pre-buffering 8192 bytes
[13:14:13.511675][stream.hls_playlist][all] #EXT-X-VERSION:3
[13:14:13.511825][stream.hls_playlist][all] #EXT-X-TARGETDURATION:8
[13:14:13.511903][stream.hls_playlist][all] #EXT-X-MEDIA-SEQUENCE:593337
[13:14:13.511969][stream.hls_playlist][all] #EXTINF:8.000000,
[13:14:13.512038][stream.hls_playlist][all] kanald_2023_04_17_11_49_07_593337.ts?live=true&app=com.kanald&min=0&max=1250
[13:14:13.512150][stream.hls_playlist][all] #EXTINF:8.000000,
[13:14:13.512224][stream.hls_playlist][all] kanald_2023_04_17_11_49_15_593338.ts?live=true&app=com.kanald&min=0&max=1250
[13:14:13.512323][stream.hls_playlist][all] #EXTINF:8.000000,
[13:14:13.512392][stream.hls_playlist][all] kanald_2023_04_17_11_49_23_593339.ts?live=true&app=com.kanald&min=0&max=1250
[13:14:13.512481][stream.hls_playlist][all] #EXTINF:8.000000,
[13:14:13.512547][stream.hls_playlist][all] kanald_2023_04_17_11_49_31_593340.ts?live=true&app=com.kanald&min=0&max=1250
[13:14:13.512635][stream.hls_playlist][all] #EXTINF:8.000000,
[13:14:13.512707][stream.hls_playlist][all] kanald_2023_04_17_11_49_39_593341.ts?live=true&app=com.kanald&min=0&max=1250
[13:14:13.512816][stream.hls_playlist][all] #EXTINF:8.000000,
[13:14:13.512887][stream.hls_playlist][all] kanald_2023_04_17_11_49_47_593342.ts?live=true&app=com.kanald&min=0&max=1250
[13:14:13.512978][stream.hls_playlist][all] #EXTINF:8.000000,
[13:14:13.513043][stream.hls_playlist][all] kanald_2023_04_17_11_49_55_593343.ts?live=true&app=com.kanald&min=0&max=1250
[13:14:13.513133][stream.hls_playlist][all] #EXTINF:8.000000,
[13:14:13.513183][stream.hls_playlist][all] kanald_2023_04_17_11_50_03_593344.ts?live=true&app=com.kanald&min=0&max=1250
[13:14:13.513240][stream.hls_playlist][all] #EXT-X-ENDLIST
[13:14:13.513309][stream.hls][debug] First Sequence: 593337; Last Sequence: 593344
[13:14:13.513355][stream.hls][debug] Start offset: 0; Duration: None; Start Sequence: 593337; End Sequence: 593344
[13:14:13.513394][stream.hls][debug] Adding segment 593337 to queue
[13:14:13.513671][stream.hls][debug] Adding segment 593338 to queue
[13:14:13.513793][stream.hls][debug] Adding segment 593339 to queue
[13:14:13.513853][stream.hls][debug] Adding segment 593340 to queue
[13:14:13.513918][stream.hls][debug] Adding segment 593341 to queue
[13:14:13.513999][stream.hls][debug] Adding segment 593342 to queue
[13:14:13.514133][stream.hls][debug] Adding segment 593343 to queue
[13:14:13.514324][stream.hls][debug] Adding segment 593344 to queue
[13:14:13.515244][stream.segmented][debug] Closing worker thread
[13:14:17.113485][stream.hls][debug] Writing segment 593337 to output
[13:14:17.114717][stream.hls][debug] Segment 593337 complete
[13:14:17.114984][cli.output][debug] Opening subprocess: /usr/bin/vlc --input-title-format https://www.kanald.com.tr/canli-yayin -
[13:14:17.617393][cli][debug] Writing stream to output
[13:14:19.328000][stream.hls][debug] Writing segment 593338 to output
[13:14:19.328587][stream.hls][debug] Segment 593338 complete
[13:14:22.449293][stream.hls][debug] Writing segment 593339 to output
[13:14:22.450269][stream.hls][debug] Segment 593339 complete
[13:14:27.685175][stream.hls][debug] Writing segment 593340 to output
[13:14:27.686156][stream.hls][debug] Segment 593340 complete
[13:14:29.403924][stream.hls][debug] Writing segment 593341 to output
[13:14:29.404655][stream.hls][debug] Segment 593341 complete
[13:14:30.516949][stream.hls][debug] Writing segment 593342 to output
[13:14:30.517549][stream.hls][debug] Segment 593342 complete
[13:14:31.776268][stream.hls][debug] Writing segment 593343 to output
[13:14:31.777112][stream.hls][debug] Segment 593343 complete
[13:14:33.022958][stream.hls][debug] Writing segment 593344 to output
[13:14:33.023646][stream.hls][debug] Segment 593344 complete
[13:14:33.023785][stream.segmented][debug] Closing writer thread
[13:14:33.024727][cli][info] Stream ended
[13:14:33.525547][cli][info] Closing currently open stream...
stulluk ~ $
```
| 2023-04-18T12:20:36 |
||
streamlink/streamlink | 5,306 | streamlink__streamlink-5306 | [
"5203"
] | 78c319508c80a5c4cf72992e7b976d0ff741c81b | diff --git a/src/streamlink/plugins/mitele.py b/src/streamlink/plugins/mitele.py
--- a/src/streamlink/plugins/mitele.py
+++ b/src/streamlink/plugins/mitele.py
@@ -113,7 +113,12 @@ def _get_streams(self):
urls.add(update_qsd(stream["stream"], qsd, quote_via=lambda string, *_, **__: string))
for url in urls:
- yield from HLSStream.parse_variant_playlist(self.session, url, name_fmt="{pixels}_{bitrate}").items()
+ yield from HLSStream.parse_variant_playlist(
+ self.session,
+ url,
+ headers={"Origin": "https://www.mitele.es"},
+ name_fmt="{pixels}_{bitrate}",
+ ).items()
__plugin__ = Mitele
| plugins.mitele: not working anymore for all channels
### Checklist
- [X] This is a plugin issue and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest stable release
### Description
Started a few days ago. And it's not working anymore on all channels.
### Debug log
```text
Channel = Cuatro
"[cli][info] Found matching plugin mitele for URL https://www.mitele.es/directo/cuatro/
error: Unable to open URL: https://directos.mitele.es/orilinear21/live/linear21/playlist/playlist.isml/ctv.m3u8?hdnts=st=1677436035~exp=1677436214~acl=/*~hmac=abf7c67309839ee28cec8fb256dd835d76dc8067d223f9479d19b635f3c87d1c (403 Client Error: Forbidden for url: https://directos.mitele.es/orilinear21/live/linear21/playlist/playlist.isml/ctv.m3u8?hdnts=st=1677436035~exp=1677436214~acl=/*~hmac=abf7c67309839ee28cec8fb256dd835d76dc8067d223f9479d19b635f3c87d1c)"
Channel = Telecinco
"[cli][info] Found matching plugin mitele for URL https://www.mitele.es/directo/telecinco/
error: Unable to open URL: https://directos.mitele.es/orilinear01/live/linear01/main/main.isml/main.m3u8?hdnts=st=1677436216~exp=1677436396~acl=/*~hmac=5d6dc18fe9aa0ce5e287b25e425f0f14b56528fed691c559a617f1635cabbafb (403 Client Error: Forbidden for url: https://directos.mitele.es/orilinear01/live/linear01/main/main.isml/main.m3u8?hdnts=st=1677436216~exp=1677436396~acl=/*~hmac=5d6dc18fe9aa0ce5e287b25e425f0f14b56528fed691c559a617f1635cabbafb)"
etc...
```
| 2023-04-24T14:28:30 |
||
streamlink/streamlink | 5,321 | streamlink__streamlink-5321 | [
"4646"
] | 0e41130e28d80fbaf898225963c71067c8890f51 | diff --git a/src/streamlink/stream/hls.py b/src/streamlink/stream/hls.py
--- a/src/streamlink/stream/hls.py
+++ b/src/streamlink/stream/hls.py
@@ -2,6 +2,7 @@
import re
import struct
from concurrent.futures import Future
+from datetime import datetime, timedelta
from typing import Any, Dict, List, NamedTuple, Optional, Tuple, Union
from urllib.parse import urlparse
@@ -22,6 +23,7 @@
from streamlink.stream.segmented import SegmentedStreamReader, SegmentedStreamWorker, SegmentedStreamWriter
from streamlink.utils.cache import LRUCache
from streamlink.utils.formatter import Formatter
+from streamlink.utils.times import now
log = logging.getLogger(__name__)
@@ -297,6 +299,7 @@ def __init__(self, *args, **kwargs) -> None:
self.playlist_end: Optional[int] = None
self.playlist_sequence: int = -1
self.playlist_sequences: List[Sequence] = []
+ self.playlist_reload_last: datetime = now()
self.playlist_reload_time: float = 6
self.playlist_reload_time_override = self.session.options.get("hls-playlist-reload-time")
self.playlist_reload_retries = self.session.options.get("hls-playlist-reload-attempts")
@@ -412,6 +415,7 @@ def duration_to_sequence(duration: float, sequences: List[Sequence]) -> int:
return default
def iter_segments(self):
+ self.playlist_reload_last = now()
try:
self.reload_playlist()
except StreamError as err:
@@ -457,7 +461,20 @@ def iter_segments(self):
self.playlist_sequence = sequence.num + 1
- if self.wait(self.playlist_reload_time):
+ # Exclude playlist fetch+processing time from the overall playlist reload time
+ # and reload playlist in a strict time interval
+ time_completed = now()
+ time_elapsed = max(0.0, (time_completed - self.playlist_reload_last).total_seconds())
+ time_wait = max(0.0, self.playlist_reload_time - time_elapsed)
+ if self.wait(time_wait):
+ if time_wait > 0:
+ # If we had to wait, then don't call now() twice and instead reference the timestamp from before
+ # the wait() call, to prevent a shifting time offset due to the execution time.
+ self.playlist_reload_last = time_completed + timedelta(seconds=time_wait)
+ else:
+ # Otherwise, get the current time, as the reload interval already has shifted.
+ self.playlist_reload_last = now()
+
try:
self.reload_playlist()
except StreamError as err:
| diff --git a/tests/mixins/stream_hls.py b/tests/mixins/stream_hls.py
--- a/tests/mixins/stream_hls.py
+++ b/tests/mixins/stream_hls.py
@@ -8,13 +8,15 @@
import requests_mock
from streamlink import Streamlink
-from streamlink.stream.hls import HLSStream, HLSStreamWriter as _HLSStreamWriter
+from streamlink.stream.hls import HLSStream, HLSStreamWorker as _HLSStreamWorker, HLSStreamWriter as _HLSStreamWriter
from tests.testutils.handshake import Handshake
TIMEOUT_AWAIT_READ = 5
TIMEOUT_AWAIT_READ_ONCE = 5
TIMEOUT_AWAIT_WRITE = 60 # https://github.com/streamlink/streamlink/issues/3868
+TIMEOUT_AWAIT_PLAYLIST_RELOAD = 5
+TIMEOUT_AWAIT_PLAYLIST_WAIT = 5
TIMEOUT_AWAIT_CLOSE = 5
@@ -90,6 +92,23 @@ def build(self, namespace):
)
+class EventedHLSStreamWorker(_HLSStreamWorker):
+ def __init__(self, *args, **kwargs):
+ super().__init__(*args, **kwargs)
+ self.handshake_reload = Handshake()
+ self.handshake_wait = Handshake()
+ self.time_wait = None
+
+ def reload_playlist(self):
+ with self.handshake_reload():
+ return super().reload_playlist()
+
+ def wait(self, time):
+ self.time_wait = time
+ with self.handshake_wait():
+ return not self.closed
+
+
class EventedHLSStreamWriter(_HLSStreamWriter):
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
@@ -230,6 +249,16 @@ def await_close(self, timeout=TIMEOUT_AWAIT_CLOSE):
thread.join(timeout)
assert self.thread.reader.closed, "Stream reader is closed"
+ def await_playlist_reload(self, timeout=TIMEOUT_AWAIT_PLAYLIST_RELOAD) -> None:
+ worker: EventedHLSStreamWorker = self.thread.reader.worker # type: ignore[assignment]
+ assert worker.is_alive()
+ assert worker.handshake_reload.step(timeout)
+
+ def await_playlist_wait(self, timeout=TIMEOUT_AWAIT_PLAYLIST_WAIT) -> None:
+ worker: EventedHLSStreamWorker = self.thread.reader.worker # type: ignore[assignment]
+ assert worker.is_alive()
+ assert worker.handshake_wait.step(timeout)
+
# make write calls on the write-thread and wait until it has finished
def await_write(self, write_calls=1, timeout=TIMEOUT_AWAIT_WRITE) -> None:
writer: EventedHLSStreamWriter = self.thread.reader.writer # type: ignore[assignment]
diff --git a/tests/stream/test_hls.py b/tests/stream/test_hls.py
--- a/tests/stream/test_hls.py
+++ b/tests/stream/test_hls.py
@@ -1,9 +1,11 @@
import os
import typing
import unittest
+from datetime import datetime, timedelta, timezone
from threading import Event
from unittest.mock import Mock, call, patch
+import freezegun
import pytest
import requests_mock
from Crypto.Cipher import AES
@@ -13,10 +15,14 @@
from streamlink.session import Streamlink
from streamlink.stream.hls import HLSStream, HLSStreamReader, MuxedHLSStream
from streamlink.stream.hls_playlist import M3U8Parser
-from tests.mixins.stream_hls import EventedHLSStreamWriter, Playlist, Segment, Tag, TestMixinStreamHLS
+from tests.mixins.stream_hls import EventedHLSStreamWorker, EventedHLSStreamWriter, Playlist, Segment, Tag, TestMixinStreamHLS
from tests.resources import text
+EPOCH = datetime(2000, 1, 1, 0, 0, 0, 0, tzinfo=timezone.utc)
+ONE_SECOND = timedelta(seconds=1.0)
+
+
class EncryptedBase:
content: bytes
content_plain: bytes
@@ -118,12 +124,20 @@ def test_url_master(self):
assert stream.url_master == "http://mocked/master.m3u8"
-class EventedHLSReader(HLSStreamReader):
+class EventedWorkerHLSStreamReader(HLSStreamReader):
+ __worker__ = EventedHLSStreamWorker
+
+
+class EventedWriterHLSStreamReader(HLSStreamReader):
__writer__ = EventedHLSStreamWriter
-class EventedHLSStream(HLSStream):
- __reader__ = EventedHLSReader
+class EventedWorkerHLSStream(HLSStream):
+ __reader__ = EventedWorkerHLSStreamReader
+
+
+class EventedWriterHLSStream(HLSStream):
+ __reader__ = EventedWriterHLSStreamReader
@patch("streamlink.stream.hls.HLSStreamWorker.wait", Mock(return_value=True))
@@ -173,9 +187,113 @@ def test_map(self):
assert self.called(map2, once=True), "Downloads second map only once"
+class TestHLSStreamWorker(TestMixinStreamHLS, unittest.TestCase):
+ __stream__ = EventedWorkerHLSStream
+
+ OPTIONS = {"stream-timeout": 1}
+
+ def tearDown(self) -> None:
+ worker: EventedHLSStreamWorker = self.thread.reader.worker # type: ignore[assignment]
+ # don't await the handshakes on error
+ worker.handshake_wait.go()
+ worker.handshake_reload.go()
+ return super().tearDown()
+
+ def get_session(self, options=None, *args, **kwargs):
+ return super().get_session({**self.OPTIONS, **(options or {})}, *args, **kwargs)
+
+ def test_playlist_reload_offset(self) -> None:
+ thread, segments = self.subject(
+ start=False,
+ playlists=[
+ Playlist(0, targetduration=5, segments=[Segment(0)]),
+ Playlist(1, targetduration=5, segments=[Segment(1)]),
+ Playlist(2, targetduration=5, segments=[Segment(2)]),
+ Playlist(3, targetduration=5, segments=[Segment(3)]),
+ Playlist(4, targetduration=5, segments=[Segment(4)], end=True),
+ ],
+ )
+ worker: EventedHLSStreamWorker = thread.reader.worker
+ targetduration = ONE_SECOND * 5
+
+ with freezegun.freeze_time(EPOCH) as frozen_time:
+ self.start()
+
+ assert worker.handshake_reload.wait_ready(1), "Arrives at initial playlist reload"
+ assert worker.playlist_reload_last == EPOCH, "Sets the initial value of the last reload time"
+
+ # adjust clock and reload playlist: let it take one second
+ frozen_time.move_to(worker.playlist_reload_last + ONE_SECOND)
+ self.await_playlist_reload()
+ assert worker.playlist_reload_time == 5.0, "Uses the playlist's targetduration as reload time"
+
+ # time_completed = 00:00:01; time_elapsed = 1s
+ assert worker.handshake_wait.wait_ready(1), "Arrives at first wait() call"
+ assert worker.playlist_sequence == 1, "Has queued first segment"
+ assert worker.time_wait == 4.0, "Waits for 4 seconds out of the 5 seconds reload time"
+ self.await_playlist_wait()
+
+ assert worker.handshake_reload.wait_ready(1), "Arrives at second playlist reload"
+ assert worker.playlist_reload_last == EPOCH + targetduration, \
+ "Last reload time is the sum of reload+wait time (=targetduration)"
+
+ # adjust clock and reload playlist: let it exceed targetduration by two seconds
+ frozen_time.move_to(worker.playlist_reload_last + targetduration + ONE_SECOND * 2)
+ self.await_playlist_reload()
+ assert worker.playlist_reload_time == 5.0, "Uses the playlist's targetduration as reload time"
+
+ # time_completed = 00:00:12; time_elapsed = 7s (exceeded 5s targetduration)
+ assert worker.handshake_wait.wait_ready(1), "Arrives at second wait() call"
+ assert worker.playlist_sequence == 2, "Has queued second segment"
+ assert worker.time_wait == 0.0, "Doesn't wait when reloading took too long"
+ self.await_playlist_wait()
+
+ assert worker.handshake_reload.wait_ready(1), "Arrives at third playlist reload"
+ assert worker.playlist_reload_last == EPOCH + targetduration * 2 + ONE_SECOND * 2, \
+ "Sets last reload time to current time when reloading took too long (changes the interval)"
+
+ # adjust clock and reload playlist: let it take one second again
+ frozen_time.move_to(worker.playlist_reload_last + ONE_SECOND)
+ self.await_playlist_reload()
+ assert worker.playlist_reload_time == 5.0, "Uses the playlist's targetduration as reload time"
+
+ # time_completed = 00:00:13; time_elapsed = 1s
+ assert worker.handshake_wait.wait_ready(1), "Arrives at third wait() call"
+ assert worker.playlist_sequence == 3, "Has queued third segment"
+ assert worker.time_wait == 4.0, "Waits for 4 seconds out of the 5 seconds reload time"
+ self.await_playlist_wait()
+
+ assert worker.handshake_reload.wait_ready(1), "Arrives at fourth playlist reload"
+ assert worker.playlist_reload_last == EPOCH + targetduration * 3 + ONE_SECOND * 2, \
+ "Last reload time is the sum of reload+wait time (=targetduration) of the changed interval"
+
+ # adjust clock and reload playlist: simulate no fetch+processing delay
+ frozen_time.move_to(worker.playlist_reload_last)
+ self.await_playlist_reload()
+ assert worker.playlist_reload_time == 5.0, "Uses the playlist's targetduration as reload time"
+
+ # time_completed = 00:00:17; time_elapsed = 0s
+ assert worker.handshake_wait.wait_ready(1), "Arrives at fourth wait() call"
+ assert worker.playlist_sequence == 4, "Has queued fourth segment"
+ assert worker.time_wait == 5.0, "Waits for the whole reload time"
+ self.await_playlist_wait()
+
+ assert worker.handshake_reload.wait_ready(1), "Arrives at fifth playlist reload"
+ assert worker.playlist_reload_last == EPOCH + targetduration * 4 + ONE_SECOND * 2, \
+ "Last reload time is the sum of reload+wait time (no delay)"
+
+ # adjusting the clock is not needed anymore
+ self.await_playlist_reload()
+ assert self.await_read(read_all=True) == self.content(segments)
+ self.await_close()
+ assert worker.playlist_sequence == 4, "Doesn't update sequence number once ended"
+ assert not worker.handshake_wait.wait_ready(0), "Doesn't wait once ended"
+ assert not worker.handshake_reload.wait_ready(0), "Doesn't reload playlist once ended"
+
+
@patch("streamlink.stream.hls.HLSStreamWorker.wait", Mock(return_value=True))
class TestHLSStreamByterange(TestMixinStreamHLS, unittest.TestCase):
- __stream__ = EventedHLSStream
+ __stream__ = EventedWriterHLSStream
# The dummy segments in the error tests are required because the writer's run loop would otherwise continue forever
# due to the segment's future result being None (no requests result), and we can't await the end of the stream
@@ -272,7 +390,7 @@ def test_offsets(self):
@patch("streamlink.stream.hls.HLSStreamWorker.wait", Mock(return_value=True))
class TestHLSStreamEncrypted(TestMixinStreamHLS, unittest.TestCase):
- __stream__ = EventedHLSStream
+ __stream__ = EventedWriterHLSStream
def get_session(self, options=None, *args, **kwargs):
session = super().get_session(options)
@@ -572,7 +690,7 @@ def test_hls_playlist_reload_time_no_data(self):
@patch("streamlink.stream.hls.log")
@patch("streamlink.stream.hls.HLSStreamWorker.wait", Mock(return_value=True))
class TestHlsPlaylistParseErrors(TestMixinStreamHLS, unittest.TestCase):
- __stream__ = EventedHLSStream
+ __stream__ = EventedWriterHLSStream
class FakePlaylist(typing.NamedTuple):
is_master: bool = False
| stream.hls: smarter playlist reload times
The `HLSStreamWorker` is currently reloading playlists of live streams in a strict, continuous time interval. Depending on the options used ([`--hls-playlist-reload-time`](https://streamlink.github.io/cli.html#cmdoption-hls-playlist-reload-time)), the reload time is either the playlist's `targetduration` (default), or the duration of the last segment, or the sum of segment durations according to the `live-edge`, or a constant time value. Having strict and continuous playlist reload wait times causes a couple of problems.
The issue is that [HTTP request/response delays (including retries)](https://github.com/streamlink/streamlink/blob/4.2.0/src/streamlink/stream/hls.py#L283-L292), [execution delays](https://github.com/streamlink/streamlink/blob/4.2.0/src/streamlink/stream/hls.py#L383-L429) and [buffer availability wait times](https://github.com/streamlink/streamlink/blob/4.2.0/src/streamlink/stream/hls.py#L302) are [not taken into account when reloading](https://github.com/streamlink/streamlink/blob/4.2.0/src/streamlink/stream/hls.py#L425), so that over time, playlist reloads will get out-of-sync compared to the playlist's segment durations as more and more delay is added between reloads, and the point where the playlist gets reloaded gets shifted.
For most streams, this is no problem, but for low latency streams, where playlist reloads are supposed to only queue the next segment (as soon as possible), shifting the reload points is a problem because the writer's queue can become empty at some point and then it'll get filled with two segments on the following reload, which will unnecessarily introduce a streaming delay.
----
The reload times of the [`DASHStreamWorker`](https://github.com/streamlink/streamlink/blob/4.2.0/src/streamlink/stream/dash.py#L78-L87) already take execution times into consideration. The intention here is of course that [DASH segments can have an availability time](https://github.com/streamlink/streamlink/blob/4.2.0/src/streamlink/stream/dash.py#L36-L40), so being precise is important.
HLS streams however also can have segment time annotations via [`EXT-X-PROGRAM-DATE-TIME`](https://github.com/streamlink/streamlink/blob/4.2.0/src/streamlink/stream/hls_playlist.py#L355-L360). The problem though is that it's an optional tag and the time doesn't necessarily have to be precise. And since the server time is unknown and can only get determined by reading optional HTTP headers or custom playlist tags, calculating an offset to the system time is often impossible. If the time offsets of the segment times could be calculated reliably, then HLS playlist reload times could be calculated so that reloads happen as soon as possible when the next segment becomes available on the server. That would be a micro-optimization, albeit not an important one, as HLS low latency streams have prefetch segments (AHLS (draft) or custom ones (eg. Twitch)).
| 2023-05-02T11:47:59 |
|
streamlink/streamlink | 5,326 | streamlink__streamlink-5326 | [
"5324"
] | 0e762f81eab23971d1f99c3f5a8cf7389822e2f0 | diff --git a/src/streamlink/plugin/api/http_session.py b/src/streamlink/plugin/api/http_session.py
--- a/src/streamlink/plugin/api/http_session.py
+++ b/src/streamlink/plugin/api/http_session.py
@@ -1,10 +1,11 @@
-import ssl
+import re
import time
from typing import Any, Dict, Pattern, Tuple
import requests.adapters
import urllib3
from requests import PreparedRequest, Request, Session
+from requests.adapters import HTTPAdapter
from streamlink.exceptions import PluginError
from streamlink.packages.requests_file import FileAdapter
@@ -12,6 +13,7 @@
from streamlink.utils.parse import parse_json, parse_xml
+# urllib3>=2.0.0: enforce_content_length now defaults to True (keep the override for backwards compatibility)
class _HTTPResponse(urllib3.response.HTTPResponse):
def __init__(self, *args, **kwargs):
# Always enforce content length validation!
@@ -50,7 +52,9 @@ def __init__(self, *args, **kwargs):
# > normalizers should use uppercase hexadecimal digits for all percent-
# > encodings.
class Urllib3UtilUrlPercentReOverride:
- _re_percent_encoding: Pattern = urllib3.util.url.PERCENT_RE # type: ignore[attr-defined]
+ # urllib3>=2.0.0: _PERCENT_RE, urllib3<2.0.0: PERCENT_RE
+ _re_percent_encoding: Pattern \
+ = getattr(urllib3.util.url, "_PERCENT_RE", getattr(urllib3.util.url, "PERCENT_RE", re.compile(r"%[a-fA-F0-9]{2}")))
# urllib3>=1.25.8
# https://github.com/urllib3/urllib3/blame/1.25.8/src/urllib3/util/url.py#L219-L227
@@ -59,7 +63,8 @@ def subn(cls, repl: Any, string: str, count: Any = None) -> Tuple[str, int]:
return string, len(cls._re_percent_encoding.findall(string))
-urllib3.util.url.PERCENT_RE = Urllib3UtilUrlPercentReOverride # type: ignore[attr-defined]
+# urllib3>=2.0.0: _PERCENT_RE, urllib3<2.0.0: PERCENT_RE
+urllib3.util.url._PERCENT_RE = urllib3.util.url.PERCENT_RE = Urllib3UtilUrlPercentReOverride # type: ignore[attr-defined]
# requests.Request.__init__ keywords, except for "hooks"
@@ -181,12 +186,24 @@ def request(self, method, url, *args, **kwargs):
return res
-class TLSSecLevel1Adapter(requests.adapters.HTTPAdapter):
+class TLSNoDHAdapter(HTTPAdapter):
def init_poolmanager(self, *args, **kwargs):
- ctx = ssl.create_default_context()
+ ctx = urllib3.util.create_urllib3_context()
+ ctx.load_default_certs()
+ ciphers = ":".join(cipher.get("name") for cipher in ctx.get_ciphers())
+ ciphers += ":!DH"
+ ctx.set_ciphers(ciphers)
+ kwargs["ssl_context"] = ctx
+ return super().init_poolmanager(*args, **kwargs)
+
+
+class TLSSecLevel1Adapter(HTTPAdapter):
+ def init_poolmanager(self, *args, **kwargs):
+ ctx = urllib3.util.create_urllib3_context()
+ ctx.load_default_certs()
ctx.set_ciphers("DEFAULT:@SECLEVEL=1")
kwargs["ssl_context"] = ctx
return super().init_poolmanager(*args, **kwargs)
-__all__ = ["HTTPSession", "TLSSecLevel1Adapter"]
+__all__ = ["HTTPSession", "TLSNoDHAdapter", "TLSSecLevel1Adapter"]
diff --git a/src/streamlink/session.py b/src/streamlink/session.py
--- a/src/streamlink/session.py
+++ b/src/streamlink/session.py
@@ -6,13 +6,13 @@
from typing import Any, Callable, ClassVar, Dict, Iterator, Mapping, Optional, Tuple, Type
import urllib3.util.connection as urllib3_util_connection
-import urllib3.util.ssl_ as urllib3_util_ssl
+from requests.adapters import HTTPAdapter
from streamlink import __version__, plugins
from streamlink.exceptions import NoPluginError, PluginError, StreamlinkDeprecationWarning
from streamlink.logger import StreamlinkLogger
from streamlink.options import Options
-from streamlink.plugin.api.http_session import HTTPSession
+from streamlink.plugin.api.http_session import HTTPSession, TLSNoDHAdapter
from streamlink.plugin.plugin import NO_PRIORITY, NORMAL_PRIORITY, Matcher, Plugin
from streamlink.utils.l10n import Localization
from streamlink.utils.module import load_module
@@ -115,14 +115,12 @@ def _set_http_attr(self, key, value):
def _set_http_disable_dh(self, key, value):
self.set_explicit(key, value)
- default_ciphers = [
- item
- for item in urllib3_util_ssl.DEFAULT_CIPHERS.split(":") # type: ignore[attr-defined]
- if item != "!DH"
- ]
if value:
- default_ciphers.append("!DH")
- urllib3_util_ssl.DEFAULT_CIPHERS = ":".join(default_ciphers) # type: ignore[attr-defined]
+ adapter = TLSNoDHAdapter()
+ else:
+ adapter = HTTPAdapter()
+
+ self.session.http.mount("https://", adapter)
@staticmethod
def _factory_set_http_attr_key_equals_value(delimiter: str) -> Callable[["StreamlinkOptions", str, Any], None]:
| diff --git a/tests/test_session.py b/tests/test_session.py
--- a/tests/test_session.py
+++ b/tests/test_session.py
@@ -8,10 +8,12 @@
import pytest
import requests_mock
import urllib3
+from requests.adapters import HTTPAdapter
import tests.plugin
from streamlink.exceptions import NoPluginError, StreamlinkDeprecationWarning
from streamlink.plugin import HIGH_PRIORITY, LOW_PRIORITY, NO_PRIORITY, NORMAL_PRIORITY, Plugin, pluginmatcher
+from streamlink.plugin.api.http_session import TLSNoDHAdapter
from streamlink.session import Streamlink
from streamlink.stream.hls import HLSStream
from streamlink.stream.http import HTTPStream
@@ -394,19 +396,17 @@ def test_ipv4_ipv6(self, mock_urllib3_util_connection):
assert session.get_option("ipv6") is False
assert mock_urllib3_util_connection.allowed_gai_family is _original_allowed_gai_family
- @patch("streamlink.session.urllib3_util_ssl", DEFAULT_CIPHERS="foo:!bar:baz")
- def test_http_disable_dh(self, mock_urllib3_util_ssl):
+ def test_http_disable_dh(self):
session = self.subject(load_plugins=False)
- assert mock_urllib3_util_ssl.DEFAULT_CIPHERS == "foo:!bar:baz"
+ assert isinstance(session.http.adapters["https://"], HTTPAdapter)
+ assert not isinstance(session.http.adapters["https://"], TLSNoDHAdapter)
session.set_option("http-disable-dh", True)
- assert mock_urllib3_util_ssl.DEFAULT_CIPHERS == "foo:!bar:baz:!DH"
-
- session.set_option("http-disable-dh", True)
- assert mock_urllib3_util_ssl.DEFAULT_CIPHERS == "foo:!bar:baz:!DH"
+ assert isinstance(session.http.adapters["https://"], TLSNoDHAdapter)
session.set_option("http-disable-dh", False)
- assert mock_urllib3_util_ssl.DEFAULT_CIPHERS == "foo:!bar:baz"
+ assert isinstance(session.http.adapters["https://"], HTTPAdapter)
+ assert not isinstance(session.http.adapters["https://"], TLSNoDHAdapter)
class TestSessionOptionHttpProxy:
| AttributeError: module 'urllib3.util.url' has no attribute 'PERCENT_RE'
### Checklist
- [X] This is a bug report and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed bug reports](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22bug%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest stable release
### Description
C:\Program Files\Python310\lib\site-packages\streamlink\plugin\api\http_session.py line 53 typo?
I was trying to run my script with python 3.10.4 in Windows 10 and got that error, but trying to run it with python 3.8.6 is working fine, why?
### Debug log
```text
File "C:\Program Files\Python310\lib\site-packages\streamlink\plugin\api\http_session.py", line 53, in Urllib3UtilUrlPercentReOverride
_re_percent_encoding: Pattern = urllib3.util.url.PERCENT_RE # type: ignore[attr-defined]
AttributeError: module 'urllib3.util.url' has no attribute 'PERCENT_RE'. Did you mean: '_PERCENT_RE'?
```
| Streamlink applies an override for a faulty url encoding in urllib3, as you can see here:
- https://github.com/streamlink/streamlink/blob/5.4.0/src/streamlink/plugin/api/http_session.py#L41-L62
- https://github.com/streamlink/streamlink/blob/5.4.0/tests/test_api_http_session.py#L12-L33
Apparently, urllib3 has renamed its `PERCENT_RE` constant to `_PERCENT_RE` in its `2.0.0` release:
https://github.com/urllib3/urllib3/blame/2.0.0/src/urllib3/util/url.py#L15
**On the 1.x branch, it's still `PERCENT_RE`:**
https://github.com/urllib3/urllib3/blame/1.26.15/src/urllib3/util/url.py#L17
**Streamlink does only support urllib3 1.x, so if you've installed urllib3 2.x in your python environment, then you're ignoring the dependency version constraints of streamlink and requests:**
- streamlink: https://github.com/streamlink/streamlink/blob/5.4.0/setup.cfg#L49-L50
- requests: https://github.com/psf/requests/blob/v2.30.0/setup.cfg#L10
Not a bug. Fix your Streamlink install / python environment.
ok, thanks
Use a virtual environment if you have other packages in your python environment which depend on urllib3 2.x.
https://streamlink.github.io/install.html#virtual-environment
So apparently `requests` has published version `2.30.0` with a bump to `urllib3` `<3`:
https://github.com/psf/requests/releases/tag/v2.30.0
I unfortunately looked at the wrong package metadata yesterday. The right one is of course here:
https://github.com/psf/requests/blame/v2.30.0/setup.py#L64
They added a changelog just now after tagging the release yesterday already. For some reason, pip didn't pick up the new version for me when I ran `pip install -U --upgrade-strategy=eager -r dev-requirements.txt -r docs-requirements.txt` yesterday after seeing this thread here.
----
This means that Streamlink has to fix the new version incompabilities now.
I've restricted urllib3 to `<2` for now in #5325, but I'm going to bump that to `<3` again with the necessary changes.
This is a bit annoying, because `requests` should've bumped its major version in order to stay compliant with the semantic versioning scheme.
Speaking of semver, urllib3 isn't using this scheme correctly either, with lots of deprecations being added in 2.0.0, while stuff will get removed/changed in a minor release later on:
https://urllib3.readthedocs.io/en/stable/v2-migration-guide.html
So what needs to fix now is the name of the `urllib3.utils.url._PATTERN_RE` constant which Streamlink overrides, and maybe some other stuff which I'm going to look at now (TLS stuff / network interface stuff).
Reopening for visibility. Once fixed, there will be a new release, since the current stable release is broken when not limiting `urllib3` to `<2`.
> For some reason, pip didn't pick up the new version for me
They dropped 2.30.0 and re-released it today:
https://github.com/psf/requests/issues/6432#issuecomment-1533483200 | 2023-05-04T16:25:49 |
streamlink/streamlink | 5,330 | streamlink__streamlink-5330 | [
"2198"
] | 25efd36928d70748add72b840d4f752b0561cde7 | diff --git a/src/streamlink/stream/hls.py b/src/streamlink/stream/hls.py
--- a/src/streamlink/stream/hls.py
+++ b/src/streamlink/stream/hls.py
@@ -292,13 +292,17 @@ class HLSStreamWorker(SegmentedStreamWorker):
writer: "HLSStreamWriter"
stream: "HLSStream"
+ SEGMENT_QUEUE_TIMING_THRESHOLD_FACTOR = 2
+
def __init__(self, *args, **kwargs) -> None:
super().__init__(*args, **kwargs)
self.playlist_changed = False
self.playlist_end: Optional[int] = None
+ self.playlist_targetduration: float = 0
self.playlist_sequence: int = -1
self.playlist_sequences: List[Sequence] = []
+ self.playlist_sequences_last: datetime = now()
self.playlist_reload_last: datetime = now()
self.playlist_reload_time: float = 6
self.playlist_reload_time_override = self.session.options.get("hls-playlist-reload-time")
@@ -353,6 +357,7 @@ def reload_playlist(self):
sequences = [Sequence(media_sequence + i, s)
for i, s in enumerate(playlist.segments)]
+ self.playlist_targetduration = playlist.targetduration or 0
self.playlist_reload_time = self._playlist_reload_time(playlist, sequences)
if sequences:
@@ -365,8 +370,8 @@ def _playlist_reload_time(self, playlist: M3U8, sequences: List[Sequence]) -> fl
return sum(s.segment.duration for s in sequences[-max(1, self.live_edge - 1):])
if type(self.playlist_reload_time_override) is float and self.playlist_reload_time_override > 0:
return self.playlist_reload_time_override
- if playlist.target_duration:
- return playlist.target_duration
+ if playlist.targetduration:
+ return playlist.targetduration
if sequences:
return sum(s.segment.duration for s in sequences[-max(1, self.live_edge - 1):])
@@ -398,6 +403,14 @@ def process_sequences(self, playlist: M3U8, sequences: List[Sequence]) -> None:
def valid_sequence(self, sequence: Sequence) -> bool:
return sequence.num >= self.playlist_sequence
+ def _segment_queue_timing_threshold_reached(self) -> bool:
+ threshold = self.playlist_targetduration * self.SEGMENT_QUEUE_TIMING_THRESHOLD_FACTOR
+ if now() <= self.playlist_sequences_last + timedelta(seconds=threshold):
+ return False
+
+ log.warning(f"No new segments in playlist for more than {threshold:.2f}s. Stopping...")
+ return True
+
@staticmethod
def duration_to_sequence(duration: float, sequences: List[Sequence]) -> int:
d = 0.0
@@ -415,7 +428,10 @@ def duration_to_sequence(duration: float, sequences: List[Sequence]) -> int:
return default
def iter_segments(self):
- self.playlist_reload_last = now()
+ self.playlist_reload_last \
+ = self.playlist_sequences_last \
+ = now()
+
try:
self.reload_playlist()
except StreamError as err:
@@ -446,9 +462,12 @@ def iter_segments(self):
total_duration = 0
while not self.closed:
+ queued = False
for sequence in filter(self.valid_sequence, self.playlist_sequences):
log.debug(f"Adding segment {sequence.num} to queue")
yield sequence
+ queued = True
+
total_duration += sequence.segment.duration
if self.duration_limit and total_duration >= self.duration_limit:
log.info(f"Stopping stream early after {self.duration_limit}")
@@ -461,6 +480,11 @@ def iter_segments(self):
self.playlist_sequence = sequence.num + 1
+ if queued:
+ self.playlist_sequences_last = now()
+ elif self._segment_queue_timing_threshold_reached():
+ return
+
# Exclude playlist fetch+processing time from the overall playlist reload time
# and reload playlist in a strict time interval
time_completed = now()
diff --git a/src/streamlink/stream/hls_playlist.py b/src/streamlink/stream/hls_playlist.py
--- a/src/streamlink/stream/hls_playlist.py
+++ b/src/streamlink/stream/hls_playlist.py
@@ -129,7 +129,7 @@ def __init__(self, uri: Optional[str] = None):
self.iframes_only: Optional[bool] = None # version >= 4
self.media_sequence: Optional[int] = None
self.playlist_type: Optional[str] = None
- self.target_duration: Optional[int] = None
+ self.targetduration: Optional[float] = None
self.start: Optional[Start] = None
self.version: Optional[int] = None
@@ -423,7 +423,7 @@ def parse_tag_ext_x_targetduration(self, value: str) -> None:
EXT-X-TARGETDURATION
https://datatracker.ietf.org/doc/html/rfc8216#section-4.3.3.1
"""
- self.m3u8.target_duration = int(value)
+ self.m3u8.targetduration = float(value)
def parse_tag_ext_x_media_sequence(self, value: str) -> None:
"""
| diff --git a/tests/stream/test_hls.py b/tests/stream/test_hls.py
--- a/tests/stream/test_hls.py
+++ b/tests/stream/test_hls.py
@@ -202,6 +202,77 @@ def tearDown(self) -> None:
def get_session(self, options=None, *args, **kwargs):
return super().get_session({**self.OPTIONS, **(options or {})}, *args, **kwargs)
+ def test_segment_queue_timing_threshold_reached(self) -> None:
+ thread, segments = self.subject(
+ start=False,
+ playlists=[
+ Playlist(0, targetduration=5, segments=[Segment(0)]),
+ # no EXT-X-ENDLIST, last mocked playlist response will be repreated forever
+ Playlist(0, targetduration=5, segments=[Segment(0), Segment(1)]),
+ ],
+ )
+ worker: EventedHLSStreamWorker = thread.reader.worker
+ targetduration = ONE_SECOND * 5
+
+ with freezegun.freeze_time(EPOCH) as frozen_time, \
+ patch("streamlink.stream.hls.log") as mock_log:
+ self.start()
+
+ assert worker.handshake_reload.wait_ready(1), "Loads playlist for the first time"
+ assert worker.playlist_sequence == -1, "Initial sequence number"
+ assert worker.playlist_sequences_last == EPOCH, "Sets the initial last queue time"
+
+ # first playlist reload has taken one second
+ frozen_time.tick(ONE_SECOND)
+ self.await_playlist_reload(1)
+
+ assert worker.handshake_wait.wait_ready(1), "Arrives at first wait() call"
+ assert worker.playlist_sequence == 1, "Updates the sequence number"
+ assert worker.playlist_sequences_last == EPOCH + ONE_SECOND, "Updates the last queue time"
+ assert worker.playlist_targetduration == 5.0
+
+ # trigger next reload when the target duration has passed
+ frozen_time.tick(targetduration)
+ self.await_playlist_wait(1)
+ self.await_playlist_reload(1)
+
+ assert worker.handshake_wait.wait_ready(1), "Arrives at second wait() call"
+ assert worker.playlist_sequence == 2, "Updates the sequence number again"
+ assert worker.playlist_sequences_last == EPOCH + ONE_SECOND + targetduration, "Updates the last queue time again"
+ assert worker.playlist_targetduration == 5.0
+
+ # trigger next reload when the target duration has passed
+ frozen_time.tick(targetduration)
+ self.await_playlist_wait(1)
+ self.await_playlist_reload(1)
+
+ assert worker.handshake_wait.wait_ready(1), "Arrives at third wait() call"
+ assert worker.playlist_sequence == 2, "Sequence number is unchanged"
+ assert worker.playlist_sequences_last == EPOCH + ONE_SECOND + targetduration, "Last queue time is unchanged"
+ assert worker.playlist_targetduration == 5.0
+
+ # trigger next reload when the target duration has passed
+ frozen_time.tick(targetduration)
+ self.await_playlist_wait(1)
+ self.await_playlist_reload(1)
+
+ assert worker.handshake_wait.wait_ready(1), "Arrives at fourth wait() call"
+ assert worker.playlist_sequence == 2, "Sequence number is unchanged"
+ assert worker.playlist_sequences_last == EPOCH + ONE_SECOND + targetduration, "Last queue time is unchanged"
+ assert worker.playlist_targetduration == 5.0
+
+ assert mock_log.warning.call_args_list == []
+
+ # trigger next reload when the target duration has passed
+ frozen_time.tick(targetduration)
+ self.await_playlist_wait(1)
+ self.await_playlist_reload(1)
+
+ self.await_read(read_all=True)
+ self.await_close(1)
+
+ assert mock_log.warning.call_args_list == [call("No new segments in playlist for more than 10.00s. Stopping...")]
+
def test_playlist_reload_offset(self) -> None:
thread, segments = self.subject(
start=False,
@@ -689,6 +760,7 @@ def test_hls_playlist_reload_time_no_data(self):
@patch("streamlink.stream.hls.log")
@patch("streamlink.stream.hls.HLSStreamWorker.wait", Mock(return_value=True))
+@patch("streamlink.stream.hls.HLSStreamWorker._segment_queue_timing_threshold_reached", Mock(return_value=False))
class TestHlsPlaylistParseErrors(TestMixinStreamHLS, unittest.TestCase):
__stream__ = EventedWriterHLSStream
diff --git a/tests/stream/test_hls_playlist.py b/tests/stream/test_hls_playlist.py
--- a/tests/stream/test_hls_playlist.py
+++ b/tests/stream/test_hls_playlist.py
@@ -381,7 +381,7 @@ def test_parse_date(self):
delta_30 = timedelta(seconds=30, milliseconds=500)
delta_60 = timedelta(seconds=60)
- assert playlist.target_duration == 120
+ assert playlist.targetduration == 120
assert list(playlist.dateranges) == [
DateRange(
| stream.hls: stop stream early when EXT-X-ENDLIST is missing
When a live stream ends, streamlink retries 12 times and then exits. (12 is a strange number and I'm not sure where it comes from. `hls-segment-attempts` is default 3, so maybe the code that uses it is being done 4 times, for a total of 12. That's just stabbing in the dark though.)
I would like to limit that to only 1 retry, because I'm worried about hitting the server too much. I was once IP banned from a server for using an automated download program, and it now makes me worry about making too many attempts-- especially attempts which are clearly automated.
Ideally, when a live stream ends, I'd like to wait 60 seconds, then retry just once. Is there a way to do that?
The message I get when a stream ends is `[stream.hls][warning] Failed to reload playlist: Unable to open URL: ... (403 Client Error: Forbidden for url:..)`, which comes from hls.py.
This is streamlink 0.14.2 on both boxes I used (Ubuntu and Windows).
---
Update: I just tried this on my Windows box, testing by turning the internet off. The first time it made 8 attempts; the second time it made 12. This was while using `--hls-playlist-reload-attempts=1`.
Update2: I actually timed the time it took to finally die and it was 38 seconds. Combined with the dead airtime before the error messages start, that's getting close to 60 seconds. Is `hls-timeout` what I need?
Update3: It looks like the time it takes can be controlled with `hls-timeout`, and these 8-12 attempts I am seeing were actually just a loop of attempts until that time runs out. So it looks like I can prevent _any_ attempts by setting that to a low value, like 15.0. That's better than nothing, although I'd still rather have it pause for a minute, then exit if it gets another 403.
| Since this was just mentioned in another thread and since I've refactored the HLS code a bit recently, what you've said regarding `--hls-timeout` (removed in favor of the generic `--stream-timeout` parameter) is correct. This parameter sets the read timeout for the output buffer in [`SegmentedStreamReader`](https://github.com/streamlink/streamlink/blob/db73cb2b00efaf54a13d6a6c642f49ea4777c6b2/src/streamlink/stream/segmented.py#L207-L236), which is 60 seconds by default. If no data was written to the buffer and there was a read attempt by the stream consumer, [this read attempt will time out](https://github.com/streamlink/streamlink/blob/master/src/streamlink/buffers.py#L104-L109) after these 60 seconds and an `OSError` will be raised with a "read timeout".
Another way of making Streamlink stop after a certain period of time when a stream has stopped but no [`#EXT-X-ENDLIST`](https://datatracker.ietf.org/doc/html/rfc8216#section-4.3.3.4) tag was set could be checking for new HLS segments to be queued. The HLSStreamWorker will continue refreshing the playlist when this tag is missing, but if there are no new segments (sequence number incrementation), this could be checked and the stream be ended this way when a certain time threshold has been reached. This would not be a graceful way of ending the stream, but the read timeout isn't either, and it's the responsibility of the server to include the endlist tag to signal the actual end of the stream.
I have a local branch here which will make Streamlink end the stream early when the HLS playlist didn't contain new segments for an X amount of time. This threshold is currently set to `TARGETDURATION * 2`.
That factor `2` is chosen very liberally, despite the HLS spec saying that the playlist's [`TARGETDURATION`](https://datatracker.ietf.org/doc/html/rfc8216#section-4.3.3.1) is the max duration of any segment, which means that when this time has passed, new segments MUST be available. The factor could maybe be reduced to 1.5 or so, but what I want to avoid are potential issues during stream discontinuities like embedded ads, where the targetduration could change to a different value that could result in the stream ending early by accident. The segment-queue timing threshold is independent of the playlist reload time since the reload time can be changed via session options and via custom stream implementations, like Twitch low latency streaming. I'm also not sure yet if I want to add a new session option that can change the value.
Since the changes depend on #5321 which is currently unmerged, I can't open a new PR for that just yet. | 2023-05-06T06:54:23 |
streamlink/streamlink | 5,333 | streamlink__streamlink-5333 | [
"5332"
] | c3258aab2c14bea7471b03e96fc1f7b5ce38b528 | diff --git a/src/streamlink/plugin/api/http_session.py b/src/streamlink/plugin/api/http_session.py
--- a/src/streamlink/plugin/api/http_session.py
+++ b/src/streamlink/plugin/api/http_session.py
@@ -13,6 +13,13 @@
from streamlink.utils.parse import parse_json, parse_xml
+try:
+ from urllib3.util import create_urllib3_context # type: ignore[attr-defined]
+except ImportError: # pragma: no cover
+ # urllib3 <2.0.0 compat import
+ from urllib3.util.ssl_ import create_urllib3_context
+
+
# urllib3>=2.0.0: enforce_content_length now defaults to True (keep the override for backwards compatibility)
class _HTTPResponse(urllib3.response.HTTPResponse):
def __init__(self, *args, **kwargs):
@@ -188,7 +195,7 @@ def request(self, method, url, *args, **kwargs):
class TLSNoDHAdapter(HTTPAdapter):
def init_poolmanager(self, *args, **kwargs):
- ctx = urllib3.util.create_urllib3_context()
+ ctx = create_urllib3_context()
ctx.load_default_certs()
ciphers = ":".join(cipher.get("name") for cipher in ctx.get_ciphers())
ciphers += ":!DH"
@@ -199,7 +206,7 @@ def init_poolmanager(self, *args, **kwargs):
class TLSSecLevel1Adapter(HTTPAdapter):
def init_poolmanager(self, *args, **kwargs):
- ctx = urllib3.util.create_urllib3_context()
+ ctx = create_urllib3_context()
ctx.load_default_certs()
ctx.set_ciphers("DEFAULT:@SECLEVEL=1")
kwargs["ssl_context"] = ctx
| TestSession.test_http_disable_dh fails with 5.5.0
### Checklist
- [X] This is a bug report and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed bug reports](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22bug%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest stable release
### Description
Since 5.5.0 TestSession.test_http_disable_dh started to fail. The urllib3 version is 1.26.15, output below under debug log.
### Debug log
```text
================================================================================= FAILURES =================================================================================
_____________________________________________________________________ TestSession.test_http_disable_dh _____________________________________________________________________
self = <tests.test_session.TestSession testMethod=test_http_disable_dh>
def test_http_disable_dh(self):
session = self.subject(load_plugins=False)
assert isinstance(session.http.adapters["https://"], HTTPAdapter)
assert not isinstance(session.http.adapters["https://"], TLSNoDHAdapter)
> session.set_option("http-disable-dh", True)
tests/test_session.py:404:
_ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _
pep517_tests_streamlink/usr/x86_64-pc-linux-gnu/lib/python3.10/site-packages/streamlink/session.py:493: in set_option
self.options.set(key, value)
pep517_tests_streamlink/usr/x86_64-pc-linux-gnu/lib/python3.10/site-packages/streamlink/options.py:54: in set
method(self, normalized, value)
pep517_tests_streamlink/usr/x86_64-pc-linux-gnu/lib/python3.10/site-packages/streamlink/session.py:119: in _set_http_disable_dh
adapter = TLSNoDHAdapter()
/usr/lib/python3.10/site-packages/requests/adapters.py:155: in __init__
self.init_poolmanager(pool_connections, pool_maxsize, block=pool_block)
_ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _
self = <streamlink.plugin.api.http_session.TLSNoDHAdapter object at 0x7f04fbe22d10>, args = (10, 10), kwargs = {'block': False}
def init_poolmanager(self, *args, **kwargs):
> ctx = urllib3.util.create_urllib3_context()
E AttributeError: module 'urllib3.util' has no attribute 'create_urllib3_context'
pep517_tests_streamlink/usr/x86_64-pc-linux-gnu/lib/python3.10/site-packages/streamlink/plugin/api/http_session.py:191: AttributeError
========================================================================= short test summary info ==========================================================================
FAILED tests/test_session.py::TestSession::test_http_disable_dh - AttributeError: module 'urllib3.util' has no attribute 'create_urllib3_context'
=============================================================== 1 failed, 5642 passed, 37 skipped in 22.51s ================================================================
```
| Yep, my bad. Our CI tests don't run on urllib3 1.26.x, so this wasn't caught. I also force-pushed a couple of times in #5326 with changes to the SSLContext initialization and then apparently didn't locally run tests again with 1.26.15.
The `create_urllib3_context` method doesn't get re-exported in the `urllib3.util.__init__` module on <2.0.0. The docs don't mention it:
- https://urllib3.readthedocs.io/en/stable/advanced-usage.html#custom-ssl-contexts
- https://github.com/urllib3/urllib3/blob/1.26.0/src/urllib3/util/__init__.py
- https://github.com/urllib3/urllib3/blob/2.0.0/src/urllib3/util/__init__.py | 2023-05-07T23:49:39 |
|
streamlink/streamlink | 5,351 | streamlink__streamlink-5351 | [
"5350"
] | c347059c4f2f2a472589a7275037f649c7920614 | diff --git a/src/streamlink/plugins/youtube.py b/src/streamlink/plugins/youtube.py
--- a/src/streamlink/plugins/youtube.py
+++ b/src/streamlink/plugins/youtube.py
@@ -135,7 +135,7 @@ def _schema_playabilitystatus(cls, data):
schema = validate.Schema(
{"playabilityStatus": {
"status": str,
- validate.optional("reason"): str,
+ validate.optional("reason"): validate.any(str, None),
}},
validate.get("playabilityStatus"),
validate.union_get("status", "reason"),
@@ -341,7 +341,8 @@ def _data_status(self, data, errorlog=False):
if not data:
return False
status, reason = self._schema_playabilitystatus(data)
- if status != "OK":
+ # assume that there's an error if reason is set (status will still be "OK" for some reason)
+ if status != "OK" or reason:
if errorlog:
log.error(f"Could not get video info - {status}: {reason}")
return False
| plugins.youtube: Checking if stream on pause or no video is playing while youtube shows it as live
### Checklist
- [X] This is a feature request and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin requests](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22feature+request%22)
### Description
I'm checking if youtube live stream is running via command:
`streamlink -Q --json --config cookie.txt https://www.youtube.com/channel/<channel_id>/live`
Everything works as expected but there is one issue:
When the streamer doesn't end live stream properly (shuts down phone, phone loosing connection, etc.), streamlink (and youtube itself) keeps showing that channel is still live.
Is there an option or any way to detect if the video is really playing? It's a bit annoying to see that someone is streaming but when I open up video, turns out there is just a "youtube's circle making rounds on video".
| Debug log with difference in couple minutes when youtube shows it as live while the video is on pause:
```
$ streamlink --loglevel=debug -Q --json --config cookie.txt https://www.youtube.com/channel/UCNcuCP5IAI6My3e5Z-vKkBQ/live
{
"plugin": "youtube",
"metadata": {
"id": "keMGL6OA6m4",
"author": "VJLinkHero",
"category": "Entertainment",
"title": "\u0412 \u043a\u0430\u043a\u0430\u0445\u0443"
},
"streams": {
"144p": {
"type": "hls",
"url": "https://manifest.googlevideo.com/api/manifest/hls_playlist/expire/1684495804/ei/XAlnZPOoIMfm1gL1zISIDg/ip/46.32.191.206/id/keMGL6OA6m4.1/itag/91/source/yt_live_broadcast/requiressl/yes/ratebypass/yes/live/1/sgoap/gir%3Dyes%3Bitag%3D139/sgovp/gir%3Dyes%3Bitag%3D160/hls_chunk_host/rr2---sn-bn251-btql.googlevideo.com/playlist_duration/30/manifest_duration/30/vprv/1/playlist_type/DVR/mh/hr/mm/44/mn/sn-bn251-btql/ms/lva/mv/u/mvi/2/pcm2cms/yes/pl/24/dover/11/pacing/0/keepalive/yes/fexp/24007246,51000022/mt/1684472925/sparams/expire,ei,ip,id,itag,source,requiressl,ratebypass,live,sgoap,sgovp,playlist_duration,manifest_duration,vprv,playlist_type/sig/AOq0QJ8wRgIhAMKP3ePcXM3v03EqI7dhbi1ERY2AEJhKnSKgkFsbIKxhAiEAmahyD4U88d0ZydSknHT07JBzKg7mtMgSxmvL0cnNTls%3D/lsparams/hls_chunk_host,mh,mm,mn,ms,mv,mvi,pcm2cms,pl/lsig/AG3C_xAwRQIhALMEOEtNZPL9KeI7DGKCdajw2kfITtXx9AkOHNxgD3a0AiAYIj3I3RE2O3R4KSY9UxcAH4aLJhp9L86sRCSaskiZXg%3D%3D/playlist/index.m3u8",
"headers": {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/112.0.0.0 Safari/537.36",
"Accept-Encoding": "gzip, deflate",
"Accept": "*/*",
"Connection": "keep-alive"
},
"master": "https://manifest.googlevideo.com/api/manifest/hls_variant/expire/1684495804/ei/XAlnZPOoIMfm1gL1zISIDg/ip/46.32.191.206/id/keMGL6OA6m4.1/source/yt_live_broadcast/requiressl/yes/hfr/1/playlist_duration/30/manifest_duration/30/maudio/1/vprv/1/go/1/pacing/0/nvgoi/1/keepalive/yes/fexp/24007246%2C51000022/dover/11/itag/0/playlist_type/DVR/sparams/expire%2Cei%2Cip%2Cid%2Csource%2Crequiressl%2Chfr%2Cplaylist_duration%2Cmanifest_duration%2Cmaudio%2Cvprv%2Cgo%2Citag%2Cplaylist_type/sig/AOq0QJ8wRQIhALvYuCrAiA-JX73OgUgC2hC9Jp3zwqPdhmZ3J8l7gBblAiA-tsmb01Jw6dA4Mba37-U8CT1fSg1vwNRPfxJF6RbZow%3D%3D/file/index.m3u8"
},
"240p": {
"type": "hls",
"url": "https://manifest.googlevideo.com/api/manifest/hls_playlist/expire/1684495804/ei/XAlnZPOoIMfm1gL1zISIDg/ip/46.32.191.206/id/keMGL6OA6m4.1/itag/92/source/yt_live_broadcast/requiressl/yes/ratebypass/yes/live/1/sgoap/gir%3Dyes%3Bitag%3D139/sgovp/gir%3Dyes%3Bitag%3D133/hls_chunk_host/rr2---sn-bn251-btql.googlevideo.com/playlist_duration/30/manifest_duration/30/vprv/1/playlist_type/DVR/mh/hr/mm/44/mn/sn-bn251-btql/ms/lva/mv/u/mvi/2/pcm2cms/yes/pl/24/dover/11/pacing/0/keepalive/yes/fexp/24007246,51000022/mt/1684472925/sparams/expire,ei,ip,id,itag,source,requiressl,ratebypass,live,sgoap,sgovp,playlist_duration,manifest_duration,vprv,playlist_type/sig/AOq0QJ8wRQIhAN4BxUXVtGdgPotil2AVIV9UvsZV1ZzfzMcbDYzrzHzKAiAun1FJCJU9EhP0OmzdROGXCVP1gYZDlndffsgqund49g%3D%3D/lsparams/hls_chunk_host,mh,mm,mn,ms,mv,mvi,pcm2cms,pl/lsig/AG3C_xAwRgIhALVYV7DKcRe3F_X1P1zOC-eDDf_ZmEDrldJFQjCzyn2MAiEAkTZD8AXRZONaWp7UfUrXe8oC5Si2bDEdluF0RbJnYQ0%3D/playlist/index.m3u8",
"headers": {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/112.0.0.0 Safari/537.36",
"Accept-Encoding": "gzip, deflate",
"Accept": "*/*",
"Connection": "keep-alive"
},
"master": "https://manifest.googlevideo.com/api/manifest/hls_variant/expire/1684495804/ei/XAlnZPOoIMfm1gL1zISIDg/ip/46.32.191.206/id/keMGL6OA6m4.1/source/yt_live_broadcast/requiressl/yes/hfr/1/playlist_duration/30/manifest_duration/30/maudio/1/vprv/1/go/1/pacing/0/nvgoi/1/keepalive/yes/fexp/24007246%2C51000022/dover/11/itag/0/playlist_type/DVR/sparams/expire%2Cei%2Cip%2Cid%2Csource%2Crequiressl%2Chfr%2Cplaylist_duration%2Cmanifest_duration%2Cmaudio%2Cvprv%2Cgo%2Citag%2Cplaylist_type/sig/AOq0QJ8wRQIhALvYuCrAiA-JX73OgUgC2hC9Jp3zwqPdhmZ3J8l7gBblAiA-tsmb01Jw6dA4Mba37-U8CT1fSg1vwNRPfxJF6RbZow%3D%3D/file/index.m3u8"
},
"360p": {
"type": "hls",
"url": "https://manifest.googlevideo.com/api/manifest/hls_playlist/expire/1684495804/ei/XAlnZPOoIMfm1gL1zISIDg/ip/46.32.191.206/id/keMGL6OA6m4.1/itag/93/source/yt_live_broadcast/requiressl/yes/ratebypass/yes/live/1/sgoap/gir%3Dyes%3Bitag%3D140/sgovp/gir%3Dyes%3Bitag%3D134/hls_chunk_host/rr2---sn-bn251-btql.googlevideo.com/playlist_duration/30/manifest_duration/30/vprv/1/playlist_type/DVR/mh/hr/mm/44/mn/sn-bn251-btql/ms/lva/mv/u/mvi/2/pcm2cms/yes/pl/24/dover/11/pacing/0/keepalive/yes/fexp/24007246,51000022/mt/1684472925/sparams/expire,ei,ip,id,itag,source,requiressl,ratebypass,live,sgoap,sgovp,playlist_duration,manifest_duration,vprv,playlist_type/sig/AOq0QJ8wRAIgXkxh1Hil1g3CqMwghcz_SWLQ1cDTZaOiyQ8r9Y4imzwCIAZzZMjjWJhzCxeaJ_eLeD_PChHAdlWQDLxA_fe5O_Wr/lsparams/hls_chunk_host,mh,mm,mn,ms,mv,mvi,pcm2cms,pl/lsig/AG3C_xAwRQIgeACTE8wEfNbNkcEZMhw-DWDlmkMhk8RH_JFYkx6TrokCIQCwmzUFtNjEAtMaMMMZovJoO7ZHoAjeB1160ZRrJ9H3EA%3D%3D/playlist/index.m3u8",
"headers": {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/112.0.0.0 Safari/537.36",
"Accept-Encoding": "gzip, deflate",
"Accept": "*/*",
"Connection": "keep-alive"
},
"master": "https://manifest.googlevideo.com/api/manifest/hls_variant/expire/1684495804/ei/XAlnZPOoIMfm1gL1zISIDg/ip/46.32.191.206/id/keMGL6OA6m4.1/source/yt_live_broadcast/requiressl/yes/hfr/1/playlist_duration/30/manifest_duration/30/maudio/1/vprv/1/go/1/pacing/0/nvgoi/1/keepalive/yes/fexp/24007246%2C51000022/dover/11/itag/0/playlist_type/DVR/sparams/expire%2Cei%2Cip%2Cid%2Csource%2Crequiressl%2Chfr%2Cplaylist_duration%2Cmanifest_duration%2Cmaudio%2Cvprv%2Cgo%2Citag%2Cplaylist_type/sig/AOq0QJ8wRQIhALvYuCrAiA-JX73OgUgC2hC9Jp3zwqPdhmZ3J8l7gBblAiA-tsmb01Jw6dA4Mba37-U8CT1fSg1vwNRPfxJF6RbZow%3D%3D/file/index.m3u8"
},
"480p": {
"type": "hls",
"url": "https://manifest.googlevideo.com/api/manifest/hls_playlist/expire/1684495804/ei/XAlnZPOoIMfm1gL1zISIDg/ip/46.32.191.206/id/keMGL6OA6m4.1/itag/94/source/yt_live_broadcast/requiressl/yes/ratebypass/yes/live/1/sgoap/gir%3Dyes%3Bitag%3D140/sgovp/gir%3Dyes%3Bitag%3D135/hls_chunk_host/rr2---sn-bn251-btql.googlevideo.com/playlist_duration/30/manifest_duration/30/vprv/1/playlist_type/DVR/mh/hr/mm/44/mn/sn-bn251-btql/ms/lva/mv/u/mvi/2/pcm2cms/yes/pl/24/dover/11/pacing/0/keepalive/yes/fexp/24007246,51000022/mt/1684472925/sparams/expire,ei,ip,id,itag,source,requiressl,ratebypass,live,sgoap,sgovp,playlist_duration,manifest_duration,vprv,playlist_type/sig/AOq0QJ8wRAIgRgn9RQgToe2Kq-zxjcfvFnCA0vH5yeE12WrA6fs9Gr8CID_Ky8RrkSOFZio1j-X_ewtXvvfe-0lNA5G4wU6_WsiN/lsparams/hls_chunk_host,mh,mm,mn,ms,mv,mvi,pcm2cms,pl/lsig/AG3C_xAwRAIgb-itzsMzGYpPEHHaC7GVln9gdsHP7gzLjp5Z1Nh8H6QCIFh8uvGOAr0MzcrBD_RgXdhqEXZX79hayaG_xfUdZ8ux/playlist/index.m3u8",
"headers": {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/112.0.0.0 Safari/537.36",
"Accept-Encoding": "gzip, deflate",
"Accept": "*/*",
"Connection": "keep-alive"
},
"master": "https://manifest.googlevideo.com/api/manifest/hls_variant/expire/1684495804/ei/XAlnZPOoIMfm1gL1zISIDg/ip/46.32.191.206/id/keMGL6OA6m4.1/source/yt_live_broadcast/requiressl/yes/hfr/1/playlist_duration/30/manifest_duration/30/maudio/1/vprv/1/go/1/pacing/0/nvgoi/1/keepalive/yes/fexp/24007246%2C51000022/dover/11/itag/0/playlist_type/DVR/sparams/expire%2Cei%2Cip%2Cid%2Csource%2Crequiressl%2Chfr%2Cplaylist_duration%2Cmanifest_duration%2Cmaudio%2Cvprv%2Cgo%2Citag%2Cplaylist_type/sig/AOq0QJ8wRQIhALvYuCrAiA-JX73OgUgC2hC9Jp3zwqPdhmZ3J8l7gBblAiA-tsmb01Jw6dA4Mba37-U8CT1fSg1vwNRPfxJF6RbZow%3D%3D/file/index.m3u8"
},
"720p": {
"type": "hls",
"url": "https://manifest.googlevideo.com/api/manifest/hls_playlist/expire/1684495804/ei/XAlnZPOoIMfm1gL1zISIDg/ip/46.32.191.206/id/keMGL6OA6m4.1/itag/95/source/yt_live_broadcast/requiressl/yes/ratebypass/yes/live/1/sgoap/gir%3Dyes%3Bitag%3D140/sgovp/gir%3Dyes%3Bitag%3D136/hls_chunk_host/rr2---sn-bn251-btql.googlevideo.com/playlist_duration/30/manifest_duration/30/vprv/1/playlist_type/DVR/mh/hr/mm/44/mn/sn-bn251-btql/ms/lva/mv/u/mvi/2/pcm2cms/yes/pl/24/dover/11/pacing/0/keepalive/yes/fexp/24007246,51000022/mt/1684472925/sparams/expire,ei,ip,id,itag,source,requiressl,ratebypass,live,sgoap,sgovp,playlist_duration,manifest_duration,vprv,playlist_type/sig/AOq0QJ8wRgIhALfc18qFLqLM8YK3TboBFMVqPFStKyQrbEKr3xpt0PAnAiEA3WNLIafe4kapoy4-MToyp3q4k8_AbQaAvXmT12Uzenc%3D/lsparams/hls_chunk_host,mh,mm,mn,ms,mv,mvi,pcm2cms,pl/lsig/AG3C_xAwRQIhAKTX4T9x6piKqJjMaYv5eOhpD8shHw59o8cZpkNxxYcnAiAiwUJmAyjnUWlaAPIoORoc0N2DGThEF5DlIEVj552cOA%3D%3D/playlist/index.m3u8",
"headers": {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/112.0.0.0 Safari/537.36",
"Accept-Encoding": "gzip, deflate",
"Accept": "*/*",
"Connection": "keep-alive"
},
"master": "https://manifest.googlevideo.com/api/manifest/hls_variant/expire/1684495804/ei/XAlnZPOoIMfm1gL1zISIDg/ip/46.32.191.206/id/keMGL6OA6m4.1/source/yt_live_broadcast/requiressl/yes/hfr/1/playlist_duration/30/manifest_duration/30/maudio/1/vprv/1/go/1/pacing/0/nvgoi/1/keepalive/yes/fexp/24007246%2C51000022/dover/11/itag/0/playlist_type/DVR/sparams/expire%2Cei%2Cip%2Cid%2Csource%2Crequiressl%2Chfr%2Cplaylist_duration%2Cmanifest_duration%2Cmaudio%2Cvprv%2Cgo%2Citag%2Cplaylist_type/sig/AOq0QJ8wRQIhALvYuCrAiA-JX73OgUgC2hC9Jp3zwqPdhmZ3J8l7gBblAiA-tsmb01Jw6dA4Mba37-U8CT1fSg1vwNRPfxJF6RbZow%3D%3D/file/index.m3u8"
},
"worst": {
"type": "hls",
"url": "https://manifest.googlevideo.com/api/manifest/hls_playlist/expire/1684495804/ei/XAlnZPOoIMfm1gL1zISIDg/ip/46.32.191.206/id/keMGL6OA6m4.1/itag/91/source/yt_live_broadcast/requiressl/yes/ratebypass/yes/live/1/sgoap/gir%3Dyes%3Bitag%3D139/sgovp/gir%3Dyes%3Bitag%3D160/hls_chunk_host/rr2---sn-bn251-btql.googlevideo.com/playlist_duration/30/manifest_duration/30/vprv/1/playlist_type/DVR/mh/hr/mm/44/mn/sn-bn251-btql/ms/lva/mv/u/mvi/2/pcm2cms/yes/pl/24/dover/11/pacing/0/keepalive/yes/fexp/24007246,51000022/mt/1684472925/sparams/expire,ei,ip,id,itag,source,requiressl,ratebypass,live,sgoap,sgovp,playlist_duration,manifest_duration,vprv,playlist_type/sig/AOq0QJ8wRgIhAMKP3ePcXM3v03EqI7dhbi1ERY2AEJhKnSKgkFsbIKxhAiEAmahyD4U88d0ZydSknHT07JBzKg7mtMgSxmvL0cnNTls%3D/lsparams/hls_chunk_host,mh,mm,mn,ms,mv,mvi,pcm2cms,pl/lsig/AG3C_xAwRQIhALMEOEtNZPL9KeI7DGKCdajw2kfITtXx9AkOHNxgD3a0AiAYIj3I3RE2O3R4KSY9UxcAH4aLJhp9L86sRCSaskiZXg%3D%3D/playlist/index.m3u8",
"headers": {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/112.0.0.0 Safari/537.36",
"Accept-Encoding": "gzip, deflate",
"Accept": "*/*",
"Connection": "keep-alive"
},
"master": "https://manifest.googlevideo.com/api/manifest/hls_variant/expire/1684495804/ei/XAlnZPOoIMfm1gL1zISIDg/ip/46.32.191.206/id/keMGL6OA6m4.1/source/yt_live_broadcast/requiressl/yes/hfr/1/playlist_duration/30/manifest_duration/30/maudio/1/vprv/1/go/1/pacing/0/nvgoi/1/keepalive/yes/fexp/24007246%2C51000022/dover/11/itag/0/playlist_type/DVR/sparams/expire%2Cei%2Cip%2Cid%2Csource%2Crequiressl%2Chfr%2Cplaylist_duration%2Cmanifest_duration%2Cmaudio%2Cvprv%2Cgo%2Citag%2Cplaylist_type/sig/AOq0QJ8wRQIhALvYuCrAiA-JX73OgUgC2hC9Jp3zwqPdhmZ3J8l7gBblAiA-tsmb01Jw6dA4Mba37-U8CT1fSg1vwNRPfxJF6RbZow%3D%3D/file/index.m3u8"
},
"best": {
"type": "hls",
"url": "https://manifest.googlevideo.com/api/manifest/hls_playlist/expire/1684495804/ei/XAlnZPOoIMfm1gL1zISIDg/ip/46.32.191.206/id/keMGL6OA6m4.1/itag/95/source/yt_live_broadcast/requiressl/yes/ratebypass/yes/live/1/sgoap/gir%3Dyes%3Bitag%3D140/sgovp/gir%3Dyes%3Bitag%3D136/hls_chunk_host/rr2---sn-bn251-btql.googlevideo.com/playlist_duration/30/manifest_duration/30/vprv/1/playlist_type/DVR/mh/hr/mm/44/mn/sn-bn251-btql/ms/lva/mv/u/mvi/2/pcm2cms/yes/pl/24/dover/11/pacing/0/keepalive/yes/fexp/24007246,51000022/mt/1684472925/sparams/expire,ei,ip,id,itag,source,requiressl,ratebypass,live,sgoap,sgovp,playlist_duration,manifest_duration,vprv,playlist_type/sig/AOq0QJ8wRgIhALfc18qFLqLM8YK3TboBFMVqPFStKyQrbEKr3xpt0PAnAiEA3WNLIafe4kapoy4-MToyp3q4k8_AbQaAvXmT12Uzenc%3D/lsparams/hls_chunk_host,mh,mm,mn,ms,mv,mvi,pcm2cms,pl/lsig/AG3C_xAwRQIhAKTX4T9x6piKqJjMaYv5eOhpD8shHw59o8cZpkNxxYcnAiAiwUJmAyjnUWlaAPIoORoc0N2DGThEF5DlIEVj552cOA%3D%3D/playlist/index.m3u8",
"headers": {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/112.0.0.0 Safari/537.36",
"Accept-Encoding": "gzip, deflate",
"Accept": "*/*",
"Connection": "keep-alive"
},
"master": "https://manifest.googlevideo.com/api/manifest/hls_variant/expire/1684495804/ei/XAlnZPOoIMfm1gL1zISIDg/ip/46.32.191.206/id/keMGL6OA6m4.1/source/yt_live_broadcast/requiressl/yes/hfr/1/playlist_duration/30/manifest_duration/30/maudio/1/vprv/1/go/1/pacing/0/nvgoi/1/keepalive/yes/fexp/24007246%2C51000022/dover/11/itag/0/playlist_type/DVR/sparams/expire%2Cei%2Cip%2Cid%2Csource%2Crequiressl%2Chfr%2Cplaylist_duration%2Cmanifest_duration%2Cmaudio%2Cvprv%2Cgo%2Citag%2Cplaylist_type/sig/AOq0QJ8wRQIhALvYuCrAiA-JX73OgUgC2hC9Jp3zwqPdhmZ3J8l7gBblAiA-tsmb01Jw6dA4Mba37-U8CT1fSg1vwNRPfxJF6RbZow%3D%3D/file/index.m3u8"
}
}
}
```
Log taken after 5 minutes of absence of stream:
```
$ streamlink --loglevel=debug -Q --json --config cookie.txt https://www.youtube.com/channel/UCNcuCP5IAI6My3e5Z-vKkBQ/live
{
"plugin": "youtube",
"metadata": {
"id": "keMGL6OA6m4",
"author": "VJLinkHero",
"category": "Entertainment",
"title": "\u0412 \u043a\u0430\u043a\u0430\u0445\u0443"
},
"streams": {
"144p": {
"type": "hls",
"url": "https://manifest.googlevideo.com/api/manifest/hls_playlist/expire/1684495912/ei/yAlnZPf3FYbWgAeBlLHwBw/ip/46.32.191.206/id/keMGL6OA6m4.1/itag/91/source/yt_live_broadcast/requiressl/yes/ratebypass/yes/live/1/sgoap/gir%3Dyes%3Bitag%3D139/sgovp/gir%3Dyes%3Bitag%3D160/hls_chunk_host/rr2---sn-bn251-btql.googlevideo.com/playlist_duration/30/manifest_duration/30/vprv/1/playlist_type/DVR/mh/hr/mm/44/mn/sn-bn251-btql/ms/lva/mv/u/mvi/2/pl/24/dover/11/pacing/0/keepalive/yes/fexp/24007246/beids/24350017/mt/1684473968/sparams/expire,ei,ip,id,itag,source,requiressl,ratebypass,live,sgoap,sgovp,playlist_duration,manifest_duration,vprv,playlist_type/sig/AOq0QJ8wRAIgIyzqP-Fxg8-6zgBLrTKqPPLU12mBfUf3R61_iTDT4mECIFEA1QFphuIb4lhPafdoiaBIr-EOAZ35TCxJ61fnLabd/lsparams/hls_chunk_host,mh,mm,mn,ms,mv,mvi,pl/lsig/AG3C_xAwRAIgNLguE-VJkd_dXpawXNhvPVSOhGiz72JL-RVwuSerVQsCIH-8U8usRI3NxAU7_s9qiUGSCJoihHRPUxOcqY-gj8CY/playlist/index.m3u8",
"headers": {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/112.0.0.0 Safari/537.36",
"Accept-Encoding": "gzip, deflate",
"Accept": "*/*",
"Connection": "keep-alive"
},
"master": "https://manifest.googlevideo.com/api/manifest/hls_variant/expire/1684495912/ei/yAlnZPf3FYbWgAeBlLHwBw/ip/46.32.191.206/id/keMGL6OA6m4.1/source/yt_live_broadcast/requiressl/yes/hfr/1/playlist_duration/30/manifest_duration/30/maudio/1/vprv/1/go/1/pacing/0/nvgoi/1/keepalive/yes/fexp/24007246/beids/24350017/dover/11/itag/0/playlist_type/DVR/sparams/expire%2Cei%2Cip%2Cid%2Csource%2Crequiressl%2Chfr%2Cplaylist_duration%2Cmanifest_duration%2Cmaudio%2Cvprv%2Cgo%2Citag%2Cplaylist_type/sig/AOq0QJ8wRgIhAIiPE0FnGEyjxLoC-1Y6zp2ynHFIPmkKyjxE7DuY8WtaAiEA9aq-A7W1wIL5nzVxg2l2oVgd455-VXVcnWrWNtFTHYk%3D/file/index.m3u8"
},
"240p": {
"type": "hls",
"url": "https://manifest.googlevideo.com/api/manifest/hls_playlist/expire/1684495912/ei/yAlnZPf3FYbWgAeBlLHwBw/ip/46.32.191.206/id/keMGL6OA6m4.1/itag/92/source/yt_live_broadcast/requiressl/yes/ratebypass/yes/live/1/sgoap/gir%3Dyes%3Bitag%3D139/sgovp/gir%3Dyes%3Bitag%3D133/hls_chunk_host/rr2---sn-bn251-btql.googlevideo.com/playlist_duration/30/manifest_duration/30/vprv/1/playlist_type/DVR/mh/hr/mm/44/mn/sn-bn251-btql/ms/lva/mv/u/mvi/2/pl/24/dover/11/pacing/0/keepalive/yes/fexp/24007246/beids/24350017/mt/1684473968/sparams/expire,ei,ip,id,itag,source,requiressl,ratebypass,live,sgoap,sgovp,playlist_duration,manifest_duration,vprv,playlist_type/sig/AOq0QJ8wRAIgGrYgWXmKdeh5HKcXry04_VUJ_csVwx93PL-sh98hNrECIDi4BoHQPDITpiQeog5V2mo1ix_ly8_FT4Txd_OmFoGd/lsparams/hls_chunk_host,mh,mm,mn,ms,mv,mvi,pl/lsig/AG3C_xAwRQIgQJGb_Lr5NltLyCokmeB01kvA7sfOTwQkBIo27x4rnAQCIQDYe8R-GjxJhhDAKHFjmwAXiCjD4QDdYE6OoWiEaRsgfg%3D%3D/playlist/index.m3u8",
"headers": {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/112.0.0.0 Safari/537.36",
"Accept-Encoding": "gzip, deflate",
"Accept": "*/*",
"Connection": "keep-alive"
},
"master": "https://manifest.googlevideo.com/api/manifest/hls_variant/expire/1684495912/ei/yAlnZPf3FYbWgAeBlLHwBw/ip/46.32.191.206/id/keMGL6OA6m4.1/source/yt_live_broadcast/requiressl/yes/hfr/1/playlist_duration/30/manifest_duration/30/maudio/1/vprv/1/go/1/pacing/0/nvgoi/1/keepalive/yes/fexp/24007246/beids/24350017/dover/11/itag/0/playlist_type/DVR/sparams/expire%2Cei%2Cip%2Cid%2Csource%2Crequiressl%2Chfr%2Cplaylist_duration%2Cmanifest_duration%2Cmaudio%2Cvprv%2Cgo%2Citag%2Cplaylist_type/sig/AOq0QJ8wRgIhAIiPE0FnGEyjxLoC-1Y6zp2ynHFIPmkKyjxE7DuY8WtaAiEA9aq-A7W1wIL5nzVxg2l2oVgd455-VXVcnWrWNtFTHYk%3D/file/index.m3u8"
},
"360p": {
"type": "hls",
"url": "https://manifest.googlevideo.com/api/manifest/hls_playlist/expire/1684495912/ei/yAlnZPf3FYbWgAeBlLHwBw/ip/46.32.191.206/id/keMGL6OA6m4.1/itag/93/source/yt_live_broadcast/requiressl/yes/ratebypass/yes/live/1/sgoap/gir%3Dyes%3Bitag%3D140/sgovp/gir%3Dyes%3Bitag%3D134/hls_chunk_host/rr2---sn-bn251-btql.googlevideo.com/playlist_duration/30/manifest_duration/30/vprv/1/playlist_type/DVR/mh/hr/mm/44/mn/sn-bn251-btql/ms/lva/mv/u/mvi/2/pl/24/dover/11/pacing/0/keepalive/yes/fexp/24007246/beids/24350017/mt/1684473968/sparams/expire,ei,ip,id,itag,source,requiressl,ratebypass,live,sgoap,sgovp,playlist_duration,manifest_duration,vprv,playlist_type/sig/AOq0QJ8wRgIhAPAHmFWjeJUG4dwFWP7ukqQk7v7Nyp_H52Kg9DFl2uBAAiEAvNPo-vSxHO2-cn6RTdjzKf6jdz8iF5x9p_Ouzxrwmak%3D/lsparams/hls_chunk_host,mh,mm,mn,ms,mv,mvi,pl/lsig/AG3C_xAwRgIhAM4VbthkBMo2NqUW-7PXfnjsV-cXgDm0NADkaM95lVJSAiEAol-5st83_EaRX70HmFa5SlUhI6LAnpu6Mga7h3Rm3Q4%3D/playlist/index.m3u8",
"headers": {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/112.0.0.0 Safari/537.36",
"Accept-Encoding": "gzip, deflate",
"Accept": "*/*",
"Connection": "keep-alive"
},
"master": "https://manifest.googlevideo.com/api/manifest/hls_variant/expire/1684495912/ei/yAlnZPf3FYbWgAeBlLHwBw/ip/46.32.191.206/id/keMGL6OA6m4.1/source/yt_live_broadcast/requiressl/yes/hfr/1/playlist_duration/30/manifest_duration/30/maudio/1/vprv/1/go/1/pacing/0/nvgoi/1/keepalive/yes/fexp/24007246/beids/24350017/dover/11/itag/0/playlist_type/DVR/sparams/expire%2Cei%2Cip%2Cid%2Csource%2Crequiressl%2Chfr%2Cplaylist_duration%2Cmanifest_duration%2Cmaudio%2Cvprv%2Cgo%2Citag%2Cplaylist_type/sig/AOq0QJ8wRgIhAIiPE0FnGEyjxLoC-1Y6zp2ynHFIPmkKyjxE7DuY8WtaAiEA9aq-A7W1wIL5nzVxg2l2oVgd455-VXVcnWrWNtFTHYk%3D/file/index.m3u8"
},
"480p": {
"type": "hls",
"url": "https://manifest.googlevideo.com/api/manifest/hls_playlist/expire/1684495912/ei/yAlnZPf3FYbWgAeBlLHwBw/ip/46.32.191.206/id/keMGL6OA6m4.1/itag/94/source/yt_live_broadcast/requiressl/yes/ratebypass/yes/live/1/sgoap/gir%3Dyes%3Bitag%3D140/sgovp/gir%3Dyes%3Bitag%3D135/hls_chunk_host/rr2---sn-bn251-btql.googlevideo.com/playlist_duration/30/manifest_duration/30/vprv/1/playlist_type/DVR/mh/hr/mm/44/mn/sn-bn251-btql/ms/lva/mv/u/mvi/2/pl/24/dover/11/pacing/0/keepalive/yes/fexp/24007246/beids/24350017/mt/1684473968/sparams/expire,ei,ip,id,itag,source,requiressl,ratebypass,live,sgoap,sgovp,playlist_duration,manifest_duration,vprv,playlist_type/sig/AOq0QJ8wRQIhAJ4KSoirqUkrCZtOng9FlC60JmSlF3HBnYU5tTxWvc5RAiBxkNzDBw4j1zee3u01-ILNsyA3D1novtUXy8E7Vmf_Dw%3D%3D/lsparams/hls_chunk_host,mh,mm,mn,ms,mv,mvi,pl/lsig/AG3C_xAwRQIhAI9jjUJsxePe8ObtxpSEbt9z0zWb6IzdQkAJiIfl8b3_AiBFPPeGzUjmOYE-pik2zRjaUVhRw_R8CWqUx1L8dDztlw%3D%3D/playlist/index.m3u8",
"headers": {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/112.0.0.0 Safari/537.36",
"Accept-Encoding": "gzip, deflate",
"Accept": "*/*",
"Connection": "keep-alive"
},
"master": "https://manifest.googlevideo.com/api/manifest/hls_variant/expire/1684495912/ei/yAlnZPf3FYbWgAeBlLHwBw/ip/46.32.191.206/id/keMGL6OA6m4.1/source/yt_live_broadcast/requiressl/yes/hfr/1/playlist_duration/30/manifest_duration/30/maudio/1/vprv/1/go/1/pacing/0/nvgoi/1/keepalive/yes/fexp/24007246/beids/24350017/dover/11/itag/0/playlist_type/DVR/sparams/expire%2Cei%2Cip%2Cid%2Csource%2Crequiressl%2Chfr%2Cplaylist_duration%2Cmanifest_duration%2Cmaudio%2Cvprv%2Cgo%2Citag%2Cplaylist_type/sig/AOq0QJ8wRgIhAIiPE0FnGEyjxLoC-1Y6zp2ynHFIPmkKyjxE7DuY8WtaAiEA9aq-A7W1wIL5nzVxg2l2oVgd455-VXVcnWrWNtFTHYk%3D/file/index.m3u8"
},
"720p": {
"type": "hls",
"url": "https://manifest.googlevideo.com/api/manifest/hls_playlist/expire/1684495912/ei/yAlnZPf3FYbWgAeBlLHwBw/ip/46.32.191.206/id/keMGL6OA6m4.1/itag/95/source/yt_live_broadcast/requiressl/yes/ratebypass/yes/live/1/sgoap/gir%3Dyes%3Bitag%3D140/sgovp/gir%3Dyes%3Bitag%3D136/hls_chunk_host/rr2---sn-bn251-btql.googlevideo.com/playlist_duration/30/manifest_duration/30/vprv/1/playlist_type/DVR/mh/hr/mm/44/mn/sn-bn251-btql/ms/lva/mv/u/mvi/2/pl/24/dover/11/pacing/0/keepalive/yes/fexp/24007246/beids/24350017/mt/1684473968/sparams/expire,ei,ip,id,itag,source,requiressl,ratebypass,live,sgoap,sgovp,playlist_duration,manifest_duration,vprv,playlist_type/sig/AOq0QJ8wRAIgQeUrPrOTPNjZOZCq29Z5bVsValW1rvGaxvU8inF-5qYCIBwsKLRgqBvIWH9Bdhua9zQCkpu1OEJYYL80tzApU4mX/lsparams/hls_chunk_host,mh,mm,mn,ms,mv,mvi,pl/lsig/AG3C_xAwRQIhAPFxP5meouDp2XYtjdup4iStawEEduWwzB2BLBfPETINAiBjtskf4gJYh85IpVW1P_3HtRsOHGdo9hOSJQgdJSTbTA%3D%3D/playlist/index.m3u8",
"headers": {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/112.0.0.0 Safari/537.36",
"Accept-Encoding": "gzip, deflate",
"Accept": "*/*",
"Connection": "keep-alive"
},
"master": "https://manifest.googlevideo.com/api/manifest/hls_variant/expire/1684495912/ei/yAlnZPf3FYbWgAeBlLHwBw/ip/46.32.191.206/id/keMGL6OA6m4.1/source/yt_live_broadcast/requiressl/yes/hfr/1/playlist_duration/30/manifest_duration/30/maudio/1/vprv/1/go/1/pacing/0/nvgoi/1/keepalive/yes/fexp/24007246/beids/24350017/dover/11/itag/0/playlist_type/DVR/sparams/expire%2Cei%2Cip%2Cid%2Csource%2Crequiressl%2Chfr%2Cplaylist_duration%2Cmanifest_duration%2Cmaudio%2Cvprv%2Cgo%2Citag%2Cplaylist_type/sig/AOq0QJ8wRgIhAIiPE0FnGEyjxLoC-1Y6zp2ynHFIPmkKyjxE7DuY8WtaAiEA9aq-A7W1wIL5nzVxg2l2oVgd455-VXVcnWrWNtFTHYk%3D/file/index.m3u8"
},
"worst": {
"type": "hls",
"url": "https://manifest.googlevideo.com/api/manifest/hls_playlist/expire/1684495912/ei/yAlnZPf3FYbWgAeBlLHwBw/ip/46.32.191.206/id/keMGL6OA6m4.1/itag/91/source/yt_live_broadcast/requiressl/yes/ratebypass/yes/live/1/sgoap/gir%3Dyes%3Bitag%3D139/sgovp/gir%3Dyes%3Bitag%3D160/hls_chunk_host/rr2---sn-bn251-btql.googlevideo.com/playlist_duration/30/manifest_duration/30/vprv/1/playlist_type/DVR/mh/hr/mm/44/mn/sn-bn251-btql/ms/lva/mv/u/mvi/2/pl/24/dover/11/pacing/0/keepalive/yes/fexp/24007246/beids/24350017/mt/1684473968/sparams/expire,ei,ip,id,itag,source,requiressl,ratebypass,live,sgoap,sgovp,playlist_duration,manifest_duration,vprv,playlist_type/sig/AOq0QJ8wRAIgIyzqP-Fxg8-6zgBLrTKqPPLU12mBfUf3R61_iTDT4mECIFEA1QFphuIb4lhPafdoiaBIr-EOAZ35TCxJ61fnLabd/lsparams/hls_chunk_host,mh,mm,mn,ms,mv,mvi,pl/lsig/AG3C_xAwRAIgNLguE-VJkd_dXpawXNhvPVSOhGiz72JL-RVwuSerVQsCIH-8U8usRI3NxAU7_s9qiUGSCJoihHRPUxOcqY-gj8CY/playlist/index.m3u8",
"headers": {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/112.0.0.0 Safari/537.36",
"Accept-Encoding": "gzip, deflate",
"Accept": "*/*",
"Connection": "keep-alive"
},
"master": "https://manifest.googlevideo.com/api/manifest/hls_variant/expire/1684495912/ei/yAlnZPf3FYbWgAeBlLHwBw/ip/46.32.191.206/id/keMGL6OA6m4.1/source/yt_live_broadcast/requiressl/yes/hfr/1/playlist_duration/30/manifest_duration/30/maudio/1/vprv/1/go/1/pacing/0/nvgoi/1/keepalive/yes/fexp/24007246/beids/24350017/dover/11/itag/0/playlist_type/DVR/sparams/expire%2Cei%2Cip%2Cid%2Csource%2Crequiressl%2Chfr%2Cplaylist_duration%2Cmanifest_duration%2Cmaudio%2Cvprv%2Cgo%2Citag%2Cplaylist_type/sig/AOq0QJ8wRgIhAIiPE0FnGEyjxLoC-1Y6zp2ynHFIPmkKyjxE7DuY8WtaAiEA9aq-A7W1wIL5nzVxg2l2oVgd455-VXVcnWrWNtFTHYk%3D/file/index.m3u8"
},
"best": {
"type": "hls",
"url": "https://manifest.googlevideo.com/api/manifest/hls_playlist/expire/1684495912/ei/yAlnZPf3FYbWgAeBlLHwBw/ip/46.32.191.206/id/keMGL6OA6m4.1/itag/95/source/yt_live_broadcast/requiressl/yes/ratebypass/yes/live/1/sgoap/gir%3Dyes%3Bitag%3D140/sgovp/gir%3Dyes%3Bitag%3D136/hls_chunk_host/rr2---sn-bn251-btql.googlevideo.com/playlist_duration/30/manifest_duration/30/vprv/1/playlist_type/DVR/mh/hr/mm/44/mn/sn-bn251-btql/ms/lva/mv/u/mvi/2/pl/24/dover/11/pacing/0/keepalive/yes/fexp/24007246/beids/24350017/mt/1684473968/sparams/expire,ei,ip,id,itag,source,requiressl,ratebypass,live,sgoap,sgovp,playlist_duration,manifest_duration,vprv,playlist_type/sig/AOq0QJ8wRAIgQeUrPrOTPNjZOZCq29Z5bVsValW1rvGaxvU8inF-5qYCIBwsKLRgqBvIWH9Bdhua9zQCkpu1OEJYYL80tzApU4mX/lsparams/hls_chunk_host,mh,mm,mn,ms,mv,mvi,pl/lsig/AG3C_xAwRQIhAPFxP5meouDp2XYtjdup4iStawEEduWwzB2BLBfPETINAiBjtskf4gJYh85IpVW1P_3HtRsOHGdo9hOSJQgdJSTbTA%3D%3D/playlist/index.m3u8",
"headers": {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/112.0.0.0 Safari/537.36",
"Accept-Encoding": "gzip, deflate",
"Accept": "*/*",
"Connection": "keep-alive"
},
"master": "https://manifest.googlevideo.com/api/manifest/hls_variant/expire/1684495912/ei/yAlnZPf3FYbWgAeBlLHwBw/ip/46.32.191.206/id/keMGL6OA6m4.1/source/yt_live_broadcast/requiressl/yes/hfr/1/playlist_duration/30/manifest_duration/30/maudio/1/vprv/1/go/1/pacing/0/nvgoi/1/keepalive/yes/fexp/24007246/beids/24350017/dover/11/itag/0/playlist_type/DVR/sparams/expire%2Cei%2Cip%2Cid%2Csource%2Crequiressl%2Chfr%2Cplaylist_duration%2Cmanifest_duration%2Cmaudio%2Cvprv%2Cgo%2Citag%2Cplaylist_type/sig/AOq0QJ8wRgIhAIiPE0FnGEyjxLoC-1Y6zp2ynHFIPmkKyjxE7DuY8WtaAiEA9aq-A7W1wIL5nzVxg2l2oVgd455-VXVcnWrWNtFTHYk%3D/file/index.m3u8"
}
}
}
```
> (and youtube itself) keeps showing that channel is still live
There's a problem with YouTube's returned metadata. Here's the relevant stuff:
```json
{
"playabilityStatus": {
"status": "OK",
"reason": "Es gibt ein technisches Problem.",
"playableInEmbed": true,
"liveStreamability": {
"liveStreamabilityRenderer": {
"videoId": "keMGL6OA6m4",
"broadcastId": "1",
"pollDelayMs": "15000"
}
},
"miniplayer": {
"miniplayerRenderer": {
"playbackMode": "PLAYBACK_MODE_ALLOW"
}
},
"contextParams": "Q0FFU0FnZ0M="
},
"videoDetails": {
"videoId": "keMGL6OA6m4",
"title": "...",
"lengthSeconds": "0",
"isLive": true,
"channelId": "UCNcuCP5IAI6My3e5Z-vKkBQ",
"isOwnerViewing": false,
"shortDescription": "...",
"isCrawlable": true,
"isLiveDvrEnabled": true,
"thumbnail": {...},
"liveChunkReadahead": 3,
"allowRatings": true,
"viewCount": "13757",
"author": "VJLinkHero",
"isLowLatencyLiveStream": true,
"isPrivate": false,
"isUnpluggedCorpus": false,
"latencyClass": "MDE_STREAM_OPTIMIZATIONS_RENDERER_LATENCY_LOW",
"isLiveContent": true
}
}
```
As you can see, the `playabilityStatus.status` value is `OK`. This is checked by Streamlink's YouTube plugin:
https://github.com/streamlink/streamlink/blob/5.5.1/src/streamlink/plugins/youtube.py#L340-L348
However, the `playabilityStatus.reason` value, at least when requested with a German IP, is `Es gibt ein technisches Problem.`, which means "There's a technical problem.". The `playabilityStatus.status` value should not be `OK` in this case. Interpreting this kind of error is a bit awkward in this situation. Checking the reason string value in different languages is obviously not a solution, so only known valid reason string values need to checked and compared against. The reason is set to `None` on valid live streams and videos though (those I've checked).
The HLS stream URLs themselves are totally fine here. What's happening is that no new HLS segments get added to the playlist, so the stream will time out eventually (`--stream-timeout` default value of 60). There's an open and unmerged PR which lets the stream end early in those cases, but that's unrelated to the YouTube issue and just an HLS playlist polling optimization. #5330
When requesting the data from the API instead of reading the embedded JSON payload, the `playabilityStatus.reason` value is `We're experiencing technical difficulties.`.
But I don't think there's any reason to check the different string values. Checking whether it's not `None` should probably be okay.
Thanks for the information.
Could you please provide command that us used to get that parameter for
checking? I'm just passing `--json` argument but I don't get
playabilityStatus parameter in output.
On Fri, May 19, 2023, 11:15 AM Sebastian Meyer ***@***.***>
wrote:
> (and youtube itself) keeps showing that channel is still live
>
> There's a problem with YouTube's returned metadata. Here's the relevant
> stuff:
>
> {
> "playabilityStatus": {
> "status": "OK",
> "reason": "Es gibt ein technisches Problem.",
> "playableInEmbed": true,
> "liveStreamability": {
> "liveStreamabilityRenderer": {
> "videoId": "keMGL6OA6m4",
> "broadcastId": "1",
> "pollDelayMs": "15000"
> }
> },
> "miniplayer": {
> "miniplayerRenderer": {
> "playbackMode": "PLAYBACK_MODE_ALLOW"
> }
> },
> "contextParams": "Q0FFU0FnZ0M="
> },
> "videoDetails": {
> "videoId": "keMGL6OA6m4",
> "title": "...",
> "lengthSeconds": "0",
> "isLive": true,
> "channelId": "UCNcuCP5IAI6My3e5Z-vKkBQ",
> "isOwnerViewing": false,
> "shortDescription": "...",
> "isCrawlable": true,
> "isLiveDvrEnabled": true,
> "thumbnail": {...},
> "liveChunkReadahead": 3,
> "allowRatings": true,
> "viewCount": "13757",
> "author": "VJLinkHero",
> "isLowLatencyLiveStream": true,
> "isPrivate": false,
> "isUnpluggedCorpus": false,
> "latencyClass": "MDE_STREAM_OPTIMIZATIONS_RENDERER_LATENCY_LOW",
> "isLiveContent": true
> }
> }
>
> As you can see, the playabilityStatus.status value is OK. This is checked
> by Streamlink's YouTube plugin:
>
> https://github.com/streamlink/streamlink/blob/5.5.1/src/streamlink/plugins/youtube.py#L340-L348
>
> However, the playabilityStatus.reason value, at least when requested with
> a German IP, is Es gibt ein technisches Problem., which means "There's a
> technical problem.". The playabilityStatus.status value should not be OK
> in this case. Interpreting this kind of error is a bit awkward in this
> situation. Checking the reason string value in different languages is
> obviously not a solution, so only known valid reason string values need to
> checked and compared against. The reason is set to None on valid live
> streams and videos though (those I've checked).
>
> The HLS stream URLs themselves are totally fine here. What's happening is
> that no new HLS segments get added to the playlist, so the stream will time
> out eventually (--stream-timeout default value of 60). There's an open
> and unmerged PR which lets the stream end early in those cases, but that's
> unrelated to the YouTube issue and just an HLS playlist polling
> optimization. #5330 <https://github.com/streamlink/streamlink/pull/5330>
>
> β
> Reply to this email directly, view it on GitHub
> <https://github.com/streamlink/streamlink/issues/5350#issuecomment-1554136399>,
> or unsubscribe
> <https://github.com/notifications/unsubscribe-auth/AIL5QKJYTHBUY7MQ5QG7RX3XG4MXTANCNFSM6AAAAAAYHJ74DA>
> .
> You are receiving this because you authored the thread.Message ID:
> ***@***.***>
>
Streamlink's `--json` output is completely unrelated to any internal YouTube plugin HTTP responses.
With an improved check, the return value will simply be
```
$ streamlink 'https://www.youtube.com/channel/UCNcuCP5IAI6My3e5Z-vKkBQ/live' best
[cli][info] Found matching plugin youtube for URL https://www.youtube.com/channel/UCNcuCP5IAI6My3e5Z-vKkBQ/live
[plugins.youtube][error] Could not get video info - OK: We're experiencing technical difficulties.
error: No playable streams found on this URL: https://www.youtube.com/channel/UCNcuCP5IAI6My3e5Z-vKkBQ/live
$ streamlink -j 'https://www.youtube.com/channel/UCNcuCP5IAI6My3e5Z-vKkBQ/live' best
{
"error": "No playable streams found on this URL: https://www.youtube.com/channel/UCNcuCP5IAI6My3e5Z-vKkBQ/live"
}
```
Streamlink doesn't support plugins providing a reason why a stream lookup failed. Plugins therefore write data to the error log channel. | 2023-05-19T07:38:53 |
|
streamlink/streamlink | 5,367 | streamlink__streamlink-5367 | [
"5366"
] | 77519b12cffe5142f7b4c9d0f901ce4fac52ea0a | diff --git a/src/streamlink/plugins/dash.py b/src/streamlink/plugins/dash.py
--- a/src/streamlink/plugins/dash.py
+++ b/src/streamlink/plugins/dash.py
@@ -11,10 +11,10 @@
@pluginmatcher(re.compile(
- r"dash://(?P<url>\S+)(?:\s(?P<params>.+))?",
+ r"dash://(?P<url>\S+)(?:\s(?P<params>.+))?$",
))
@pluginmatcher(priority=LOW_PRIORITY, pattern=re.compile(
- r"(?P<url>\S+\.mpd(?:\?\S*)?)(?:\s(?P<params>.+))?",
+ r"(?P<url>\S+\.mpd(?:\?\S*)?)(?:\s(?P<params>.+))?$",
))
class MPEGDASH(Plugin):
@classmethod
diff --git a/src/streamlink/plugins/hls.py b/src/streamlink/plugins/hls.py
--- a/src/streamlink/plugins/hls.py
+++ b/src/streamlink/plugins/hls.py
@@ -11,10 +11,10 @@
@pluginmatcher(re.compile(
- r"hls(?:variant)?://(?P<url>\S+)(?:\s(?P<params>.+))?",
+ r"hls(?:variant)?://(?P<url>\S+)(?:\s(?P<params>.+))?$",
))
@pluginmatcher(priority=LOW_PRIORITY, pattern=re.compile(
- r"(?P<url>\S+\.m3u8(?:\?\S*)?)(?:\s(?P<params>.+))?",
+ r"(?P<url>\S+\.m3u8(?:\?\S*)?)(?:\s(?P<params>.+))?$",
))
class HLSPlugin(Plugin):
def _get_streams(self):
diff --git a/src/streamlink/plugins/http.py b/src/streamlink/plugins/http.py
--- a/src/streamlink/plugins/http.py
+++ b/src/streamlink/plugins/http.py
@@ -11,7 +11,7 @@
@pluginmatcher(re.compile(
- r"httpstream://(?P<url>\S+)(?:\s(?P<params>.+))?",
+ r"httpstream://(?P<url>\S+)(?:\s(?P<params>.+))?$",
))
class HTTPStreamPlugin(Plugin):
def _get_streams(self):
| diff --git a/tests/plugins/test_dash.py b/tests/plugins/test_dash.py
--- a/tests/plugins/test_dash.py
+++ b/tests/plugins/test_dash.py
@@ -11,13 +11,34 @@
class TestPluginCanHandleUrlMPEGDASH(PluginCanHandleUrl):
__plugin__ = MPEGDASH
- should_match = [
- "example.com/foo.mpd",
- "http://example.com/foo.mpd",
- "https://example.com/foo.mpd",
- "dash://example.com/foo",
- "dash://http://example.com/foo",
- "dash://https://example.com/foo",
+ should_match_groups = [
+ # implicit DASH URLs
+ ("example.com/foo.mpd", {"url": "example.com/foo.mpd"}),
+ ("example.com/foo.mpd?bar", {"url": "example.com/foo.mpd?bar"}),
+ ("http://example.com/foo.mpd", {"url": "http://example.com/foo.mpd"}),
+ ("http://example.com/foo.mpd?bar", {"url": "http://example.com/foo.mpd?bar"}),
+ ("https://example.com/foo.mpd", {"url": "https://example.com/foo.mpd"}),
+ ("https://example.com/foo.mpd?bar", {"url": "https://example.com/foo.mpd?bar"}),
+ # explicit DASH URLs with protocol prefix
+ ("dash://example.com/foo?bar", {"url": "example.com/foo?bar"}),
+ ("dash://http://example.com/foo?bar", {"url": "http://example.com/foo?bar"}),
+ ("dash://https://example.com/foo?bar", {"url": "https://example.com/foo?bar"}),
+ # optional parameters
+ ("example.com/foo.mpd?bar abc=def", {"url": "example.com/foo.mpd?bar", "params": "abc=def"}),
+ ("http://example.com/foo.mpd?bar abc=def", {"url": "http://example.com/foo.mpd?bar", "params": "abc=def"}),
+ ("https://example.com/foo.mpd?bar abc=def", {"url": "https://example.com/foo.mpd?bar", "params": "abc=def"}),
+ ("dash://https://example.com/foo?bar abc=def", {"url": "https://example.com/foo?bar", "params": "abc=def"}),
+ ]
+
+ should_not_match = [
+ # implicit DASH URLs must have their path end with ".mpd"
+ "example.com/mpd",
+ "example.com/mpd abc=def",
+ "example.com/foo.mpd,bar",
+ "example.com/foo.mpd,bar abc=def",
+ # missing parameters
+ "example.com/foo.mpd ",
+ "dash://example.com/foo ",
]
diff --git a/tests/plugins/test_hls.py b/tests/plugins/test_hls.py
--- a/tests/plugins/test_hls.py
+++ b/tests/plugins/test_hls.py
@@ -12,16 +12,39 @@
class TestPluginCanHandleUrlHLSPlugin(PluginCanHandleUrl):
__plugin__ = HLSPlugin
- should_match = [
- "example.com/foo.m3u8",
- "http://example.com/foo.m3u8",
- "https://example.com/foo.m3u8",
- "hls://example.com/foo",
- "hls://http://example.com/foo",
- "hls://https://example.com/foo",
- "hlsvariant://example.com/foo",
- "hlsvariant://http://example.com/foo",
- "hlsvariant://https://example.com/foo",
+ should_match_groups = [
+ # implicit HLS URLs
+ ("example.com/foo.m3u8", {"url": "example.com/foo.m3u8"}),
+ ("example.com/foo.m3u8?bar", {"url": "example.com/foo.m3u8?bar"}),
+ ("http://example.com/foo.m3u8", {"url": "http://example.com/foo.m3u8"}),
+ ("http://example.com/foo.m3u8?bar", {"url": "http://example.com/foo.m3u8?bar"}),
+ ("https://example.com/foo.m3u8", {"url": "https://example.com/foo.m3u8"}),
+ ("https://example.com/foo.m3u8?bar", {"url": "https://example.com/foo.m3u8?bar"}),
+ # explicit HLS URLs with protocol prefix
+ ("hls://example.com/foo?bar", {"url": "example.com/foo?bar"}),
+ ("hls://http://example.com/foo?bar", {"url": "http://example.com/foo?bar"}),
+ ("hls://https://example.com/foo?bar", {"url": "https://example.com/foo?bar"}),
+ ("hlsvariant://example.com/foo?bar", {"url": "example.com/foo?bar"}),
+ ("hlsvariant://http://example.com/foo?bar", {"url": "http://example.com/foo?bar"}),
+ ("hlsvariant://https://example.com/foo?bar", {"url": "https://example.com/foo?bar"}),
+ # optional parameters
+ ("example.com/foo.m3u8?bar abc=def", {"url": "example.com/foo.m3u8?bar", "params": "abc=def"}),
+ ("http://example.com/foo.m3u8?bar abc=def", {"url": "http://example.com/foo.m3u8?bar", "params": "abc=def"}),
+ ("https://example.com/foo.m3u8?bar abc=def", {"url": "https://example.com/foo.m3u8?bar", "params": "abc=def"}),
+ ("hls://https://example.com/foo?bar abc=def", {"url": "https://example.com/foo?bar", "params": "abc=def"}),
+ ("hlsvariant://https://example.com/foo?bar abc=def", {"url": "https://example.com/foo?bar", "params": "abc=def"}),
+ ]
+
+ should_not_match = [
+ # implicit HLS URLs must have their path end with ".m3u8"
+ "example.com/m3u8",
+ "example.com/m3u8 abc=def",
+ "example.com/foo.m3u8,bar",
+ "example.com/foo.m3u8,bar abc=def",
+ # missing parameters
+ "example.com/foo.m3u8 ",
+ "hls://example.com/foo ",
+ "hlsvariant://example.com/foo ",
]
diff --git a/tests/plugins/test_http.py b/tests/plugins/test_http.py
--- a/tests/plugins/test_http.py
+++ b/tests/plugins/test_http.py
@@ -11,10 +11,20 @@
class TestPluginCanHandleUrlHTTPStreamPlugin(PluginCanHandleUrl):
__plugin__ = HTTPStreamPlugin
- should_match = [
- "httpstream://example.com/foo",
- "httpstream://http://example.com/foo",
- "httpstream://https://example.com/foo",
+ should_match_groups = [
+ # explicit HTTPStream URLs
+ ("httpstream://example.com/foo", {"url": "example.com/foo"}),
+ ("httpstream://http://example.com/foo", {"url": "http://example.com/foo"}),
+ ("httpstream://https://example.com/foo", {"url": "https://example.com/foo"}),
+ # optional parameters
+ ("httpstream://example.com/foo abc=def", {"url": "example.com/foo", "params": "abc=def"}),
+ ("httpstream://http://example.com/foo abc=def", {"url": "http://example.com/foo", "params": "abc=def"}),
+ ("httpstream://https://example.com/foo abc=def", {"url": "https://example.com/foo", "params": "abc=def"}),
+ ]
+
+ should_not_match = [
+ # missing parameters
+ "httpstream://example.com/foo ",
]
| plugins.dash: partial URL gets matched
### Checklist
- [X] This is a bug report and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed bug reports](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22bug%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest stable release
### Description
Hello.
First of all, I must say that I'm not a programmer, I'm, at most, tech savy, so forgive if I'm making something stupid or not seing the obvious. I also tried to google the issue, but I got no results.
Recently, my provider changed the url scheme and I'm unable to get the manifests/streams using Streamlink (I would like to note that there's no DRM involved whatsoever).
Previously, my provider had links with the following scheme, that worked great:
`https://hostname/sdash/channel,token=XXXXXXXXXXXXXXXX/index.mpd?device=PC`
But now they have the following scheme:
`https://hostname/sdash/channel/index.mpd,token=XXXXXXXXXXXXXXXX/Manifest?device=PC`
This seems to be some regex problem, because Streamlink gets the link up to .mpd and seems to discard the rest of the link (it gets the "https://hostname/sdash/channel/index.mpd", but discards the ",token=XXXXXXXXXXXXXXXX/Manifest?device=PC"), making it unable to get the manifest/stream.
If I open the same https://hostname/sdash/channel/index.mpd (without the rest of the link) in the browser, I get the same error code 400. Also, I piped the same links (https://hostname/sdash/channel/index.mpd,token=XXXXXXXXXXXXXXXX/Manifest?device=PC), with the same data, through ffmpeg and it's working.
I read the cli documentation and I did't see anything that could help me to solve this, so I'm reporting this possible issue.
Lastly, I would like to thank to all Streamlink contributers, because it's an amazing piece of software.
PS: I had to redact most of the link, because it contains personaly identifiable data. The streams are also geoblocked.
### Debug log
```text
[cli][debug] OS: Linux-6.3.3-artix1-1-x86_64-with-glibc2.37
[cli][debug] Python: 3.11.3
[cli][debug] Streamlink: 5.5.1
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.5.7
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.2
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.18.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.28.2
[cli][debug] urllib3: 1.26.15
[cli][debug] websocket-client: 1.5.2
[cli][debug] Arguments:
[cli][debug] url=https://hostname/sdash/channel/index.mpd,token=XXXXXXXXXXXXXXXX/Manifest?device=PC
[cli][debug] --loglevel=debug
[cli][debug] --logfile=/home/PC/log.log
[cli][debug] --player=mpv
[cli][debug] --http-header=[('User-Agent', 'PC')]
[cli][info] Found matching plugin dash for URL https://hostname/sdash/channel/index.mpd,token=XXXXXXXXXXXXXXXX/Manifest?device=PC
[plugins.dash][debug] URL=https://hostname/sdash/channel/index.mpd; params={}
error: Unable to open URL: https://hostname/sdash/channel/index.mpd (400 Client Error: Bad Request for url: https://hostname/sdash/channel/index.mpd)
```
| > This seems to be some regex problem
No, you're just not using your command line shell correctly. It interprets `?` as a special character.
https://streamlink.github.io/cli/tutorial.html#shell-syntax
> > This seems to be some regex problem
>
> No, you're just not using your command line shell correctly. It interprets `?` as a special character. https://streamlink.github.io/cli/tutorial.html#shell-syntax
I always escape the links using single quotes.
When my provider used the previous scheme, it worked:
https://hostname/sdash/channel,token=XXXXXXXXXXXXXXXX/index.mpd?device=PC
The debug log shows the exact arguments it has received. If you get a 4xx or 5xx HTTP response, then you're missing headers or query parameters, or your access is restricted otherwise.
It's also possible that you need to set the explicit `dash://` prefix to your input URL because of how the DASH plugin receives its (implicit / low-priority) input URL.
https://github.com/streamlink/streamlink/blob/77519b12cffe5142f7b4c9d0f901ce4fac52ea0a/src/streamlink/plugins/dash.py#L13-L18
```sh
streamlink 'dash://https://...' best
```
https://streamlink.github.io/latest/cli/protocols.html#streaming-protocols | 2023-05-29T21:08:52 |
streamlink/streamlink | 5,369 | streamlink__streamlink-5369 | [
"5355"
] | 34fa502f4416531e45b02fb49a13d55f07d7efb0 | diff --git a/src/streamlink/plugins/pluzz.py b/src/streamlink/plugins/pluzz.py
--- a/src/streamlink/plugins/pluzz.py
+++ b/src/streamlink/plugins/pluzz.py
@@ -80,7 +80,7 @@ def _get_streams(self):
str,
),
validate.all(
- validate.xml_xpath_string(".//*[@data-id][@class='magneto'][1]/@data-id"),
+ validate.xml_xpath_string(".//*[@data-id][contains(@class,'magneto')][1]/@data-id"),
str,
),
),
| diff --git a/tests/plugins/test_pluzz.py b/tests/plugins/test_pluzz.py
--- a/tests/plugins/test_pluzz.py
+++ b/tests/plugins/test_pluzz.py
@@ -12,8 +12,11 @@ class TestPluginCanHandleUrlPluzz(PluginCanHandleUrl):
"https://www.france.tv/france-5/direct.html",
"https://www.france.tv/franceinfo/direct.html",
"https://www.france.tv/france-2/journal-20h00/141003-edition-du-lundi-8-mai-2017.html",
- "https://france3-regions.francetvinfo.fr/bourgogne-franche-comte/tv/direct/franche-comte",
+ "https://france3-regions.francetvinfo.fr/bourgogne-franche-comte/direct/franche-comte",
"https://www.francetvinfo.fr/en-direct/tv.html",
"https://www.francetvinfo.fr/meteo/orages/inondations-dans-le-gard-plus-de-deux-mois-de-pluie-en-quelques-heures-des"
+ "-degats-mais-pas-de-victime_4771265.html",
+ "https://la1ere.francetvinfo.fr/info-en-continu-24-24",
+ "https://la1ere.francetvinfo.fr/programme-video/france-3_outremer-ledoc/diffusion/"
+ + "2958951-polynesie-les-sages-de-l-ocean.html",
]
| plugins.pluzz: La 1ere subdomain from francetvinfo.fr is not supported
### Checklist
- [X] This is a plugin issue and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest stable release
### Description
VODs and 24/7 live from the La 1ere francetvinfo.fr subdomain (https://la1ere.francetvinfo.fr) are not supported by the Pluzz plugin. Find the original .m3u8 works for the live, but the link changes every (at least) 2 hours.
First log with VOD : https://la1ere.francetvinfo.fr/programme-video/la1ere_mitterrand-et-l-outre-mer/diffusion/2976069-espoir-et-promesses.html
Second log with live 24/7 : https://la1ere.francetvinfo.fr/info-en-continu-24-24 (HTTP referer needed for playing it)
French IP needed for playing both.
### Debug log
```text
streamlink --loglevel=debug https://la1ere.francetvinfo.fr/programme-video/la1ere_mitterrand-et-l-outre-mer/diffusion/2976069-espoir-et-promesses.html best
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.11.3
[cli][debug] Streamlink: 5.5.1
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.5.7
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.2
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.17
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.29.0
[cli][debug] urllib3: 1.26.15
[cli][debug] websocket-client: 1.5.1
[cli][debug] Arguments:
[cli][debug] url=https://la1ere.francetvinfo.fr/programme-video/la1ere_mitterrand-et-l-outre-mer/diffusion/2976069-espoir-et-promesses.html
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --player="D:\Program Files\VideoLAN\VLC\vlc.exe" --file-caching=5000
[cli][debug] --ffmpeg-ffmpeg=C:\Users\Orion\AppData\Local\Programs\Streamlink\ffmpeg\ffmpeg.exe
[cli][info] Found matching plugin pluzz for URL https://la1ere.francetvinfo.fr/programme-video/la1ere_mitterrand-et-l-outre-mer/diffusion/2976069-espoir-et-promesses.html
[plugins.pluzz][debug] Country: FR
error: No playable streams found on this URL: https://la1ere.francetvinfo.fr/programme-video/la1ere_mitterrand-et-l-outre-mer/diffusion/2976069-espoir-et-promesses.html
streamlink --loglevel=debug --http-header "Referer= https://la1ere.francetvinfo.fr" https://la1ere.francetvinfo.fr/info-en-continu-24-24 best
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.11.3
[cli][debug] Streamlink: 5.5.1
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.5.7
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.2
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.17
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.29.0
[cli][debug] urllib3: 1.26.15
[cli][debug] websocket-client: 1.5.1
[cli][debug] Arguments:
[cli][debug] url=https://la1ere.francetvinfo.fr/info-en-continu-24-24
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --player="D:\Program Files\VideoLAN\VLC\vlc.exe" --file-caching=5000
[cli][debug] --ffmpeg-ffmpeg=C:\Users\Orion\AppData\Local\Programs\Streamlink\ffmpeg\ffmpeg.exe
[cli][debug] --http-header=[('Referer', 'https://la1ere.francetvinfo.fr')]
[cli][info] Found matching plugin pluzz for URL https://la1ere.francetvinfo.fr/info-en-continu-24-24
[plugins.pluzz][debug] Country: FR
error: No playable streams found on this URL: https://la1ere.francetvinfo.fr/info-en-continu-24-24
```
| 2023-05-31T12:04:03 |
|
streamlink/streamlink | 5,374 | streamlink__streamlink-5374 | [
"5365"
] | ef7165d576d1ed7e31ffdeef079b4f7ef512c216 | diff --git a/src/streamlink/plugins/turkuvaz.py b/src/streamlink/plugins/turkuvaz.py
--- a/src/streamlink/plugins/turkuvaz.py
+++ b/src/streamlink/plugins/turkuvaz.py
@@ -10,58 +10,85 @@
$url minikacocuk.com.tr
$url minikago.com.tr
$url vavtv.com.tr
-$type live
+$type live, vod
$region various
"""
+import logging
import re
-from urllib.parse import urlparse
from streamlink.plugin import Plugin, pluginmatcher
from streamlink.plugin.api import validate
from streamlink.stream.hls import HLSStream
+log = logging.getLogger(__name__)
+
+
@pluginmatcher(re.compile(r"""
https?://(?:www\.)?
(?:
- (?:
- atvavrupa\.tv
- |
- (?:atv|a2tv|ahaber|aspor|minikago|minikacocuk|anews)\.com\.tr
- )/webtv/(?:live-broadcast|canli-yayin)
- |
- ahaber\.com\.tr/video/canli-yayin
+ atvavrupa\.tv
|
- (?:atv|apara|vavtv)\.com\.tr/canli-yayin
- |
- atv\.com\.tr/a2tv/canli-yayin
+ (?:a2tv|ahaber|anews|apara|aspor|atv|minikacocuk|minikago|vavtv)\.com\.tr
)
""", re.VERBOSE))
class Turkuvaz(Plugin):
- _URL_HLS = "https://trkvz-live.ercdn.net/{channel}/{channel}.m3u8"
- _URL_TOKEN = "https://securevideotoken.tmgrup.com.tr/webtv/secure"
+ def _get_streams(self):
+ _find_and_get_attrs = validate.Schema(
+ validate.xml_find(".//div[@data-videoid][@data-websiteid]"),
+ validate.union_get("data-videoid", "data-websiteid"),
+ )
- _MAP_HOST_TO_CHANNEL = {
- "atv": "atvhd",
- "ahaber": "ahaberhd",
- "apara": "aparahd",
- "aspor": "asporhd",
- "anews": "anewshd",
- "vavtv": "vavtv",
- "minikacocuk": "minikagococuk",
- }
+ id_data = self.session.http.get(
+ self.url,
+ schema=validate.Schema(
+ validate.parse_html(),
+ validate.any(
+ _find_and_get_attrs,
+ validate.all(
+ validate.xml_xpath_string(
+ ".//script[contains(text(),'data-videoid') and contains(text(),'data-websiteid')]/text()",
+ ),
+ validate.none_or_all(
+ str,
+ validate.regex(re.compile(r"""var\s+tmdPlayer\s*=\s*(?P<q>["'])(.*?)(?P=q)""")),
+ validate.get(0),
+ validate.parse_html(),
+ _find_and_get_attrs,
+ ),
+ ),
+ ),
+ ),
+ )
- def _get_streams(self):
- hostname = re.sub(r"^www\.", "", urlparse(self.url).netloc).split(".")[0]
+ if not id_data:
+ return
+
+ video_id, website_id = id_data
+ log.debug(f"video_id={video_id}")
+ log.debug(f"website_id={website_id}")
- # remap the domain to channel
- channel = self._MAP_HOST_TO_CHANNEL.get(hostname, hostname)
- hls_url = self._URL_HLS.format(channel=channel)
+ self.id, self.title, hls_url = self.session.http.get(
+ f"https://videojs.tmgrup.com.tr/getvideo/{website_id}/{video_id}",
+ schema=validate.Schema(
+ validate.parse_json(),
+ {
+ "success": True,
+ "video": {
+ "VideoId": str,
+ "Title": str,
+ "VideoSmilUrl": validate.url(),
+ },
+ },
+ validate.get("video"),
+ validate.union_get("VideoId", "Title", "VideoSmilUrl"),
+ ),
+ )
+ log.debug(f"hls_url={hls_url}")
- # get the secure HLS URL
secure_hls_url = self.session.http.get(
- self._URL_TOKEN,
+ "https://securevideotoken.tmgrup.com.tr/webtv/secure",
params=f"url={hls_url}",
headers={"Referer": self.url},
schema=validate.Schema(
@@ -73,6 +100,7 @@ def _get_streams(self):
validate.get("Url"),
),
)
+ log.debug(f"secure_hls_url={secure_hls_url}")
if secure_hls_url:
return HLSStream.parse_variant_playlist(self.session, secure_hls_url)
| diff --git a/tests/plugins/test_turkuvaz.py b/tests/plugins/test_turkuvaz.py
--- a/tests/plugins/test_turkuvaz.py
+++ b/tests/plugins/test_turkuvaz.py
@@ -6,16 +6,35 @@ class TestPluginCanHandleUrlTurkuvaz(PluginCanHandleUrl):
__plugin__ = Turkuvaz
should_match = [
- "https://www.ahaber.com.tr/video/canli-yayin",
- "https://www.ahaber.com.tr/webtv/canli-yayin",
- "https://www.anews.com.tr/webtv/live-broadcast",
- "https://www.apara.com.tr/canli-yayin",
- "https://www.aspor.com.tr/webtv/canli-yayin",
- "https://www.atv.com.tr/canli-yayin",
- "https://www.atv.com.tr/a2tv/canli-yayin",
- "https://www.atv.com.tr/webtv/canli-yayin",
- "https://www.atvavrupa.tv/webtv/canli-yayin",
- "https://www.minikacocuk.com.tr/webtv/canli-yayin",
- "https://www.minikago.com.tr/webtv/canli-yayin",
- "https://www.vavtv.com.tr/canli-yayin",
+ # canonical live links
+ "https://www.ahaber.com.tr/video/canli-yayin", # ahbr
+ "https://www.ahaber.com.tr/canli-yayin-aspor.html", # ahbr: aspor
+ "https://www.ahaber.com.tr/canli-yayin-anews.html", # ahbr: anews
+ "https://www.ahaber.com.tr/canli-yayin-a2tv.html", # ahbr: a2tv
+ "https://www.ahaber.com.tr/canli-yayin-minikago.html", # ahbr: minika go
+ "https://www.ahaber.com.tr/canli-yayin-minikacocuk.html", # ahbr: minika cocuk
+ "https://www.anews.com.tr/webtv/live-broadcast", # anews
+ "https://www.apara.com.tr/canli-yayin", # apara
+ "https://www.aspor.com.tr/webtv/canli-yayin", # aspor
+ "https://www.atv.com.tr/canli-yayin", # atv
+ "https://www.atv.com.tr/a2tv/canli-yayin", # a2tv
+ "https://www.atvavrupa.tv/canli-yayin", # atvavrupa
+ "https://www.minikacocuk.com.tr/webtv/canli-yayin", # minika cocuk
+ "https://www.minikago.com.tr/webtv/canli-yayin", # minika go
+ "https://vavtv.com.tr/canli-yayin", # vavtv
+
+ #vods
+ "https://www.ahaber.com.tr/video/yasam-videolari/samsunda-sel-sularindan-kacma-ani-kamerada",
+ "https://www.anews.com.tr/webtv/world/pro-ukraine-militia-says-it-has-captured-russian-soldiers",
+ "https://www.apara.com.tr/video/ekonomide-bugun/bist-100de-kar-satislari-derinlesir-mi",
+ "https://www.aspor.com.tr/webtv/galatasaray/galatasaraydan-forma-tanitiminda-fenerbahceye-gonderme",
+ "https://www.atv.com.tr/kurulus-osman/127-bolum/izle",
+ "https://www.atvavrupa.tv/diger-program//ozelvideo/izle",
+ "https://www.minikacocuk.com.tr/webtv/olly/bolum/olly-eylul-tanitim",
+ "https://www.minikago.com.tr/webtv/mondo-yan/bolum/mondo-yan-eylul-tanitim",
+ "https://vavtv.com.tr/vavradyo/video/guncel/munafiklarin-ozellikleri-nelerdir",
+
+ # other links for info/doc
+ "https://www.atv.com.tr/webtv/canli-yayin", # redirect to atv.com.tr/canli-yayin
+ "https://www.ahaber.com.tr/canli-yayin-atv.html", # links to atv.com.tr/webtv/canli-yayin
]
| plugins.turkuvaz: URLs outdated
### Checklist
- [X] This is a plugin issue and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest stable release
### Description
The pages for the Turkish streams like ATV and A2TV have been changed by the provider. Unlike before,now the streams are on the same page, the only difference is the directory after the domain directory,
e.g.:
https://www.atv.com.tr/canli-yayin
https://www.atv.com.tr/a2tv/canli-yayin.
Unfortunately, this means that only the stream for ATV is fetched not A2TV even though you gave the correct FQDNfor A2TV.
And ATV Avrupa is no longer recognized. you get the error message "No plugin can handle URL: https://www.atvavrupa.tv/canli-yayin" although the plugin apparently exists.
### Debug log
```text
error: No plugin can handle URL: https://www.atvavrupa.tv/canli-yayin
```
| 2023-06-05T00:59:43 |
|
streamlink/streamlink | 5,376 | streamlink__streamlink-5376 | [
"5328"
] | 28ab1e3b2e6cef7d842a8a30ba5685260659118a | diff --git a/src/streamlink/plugins/mediavitrina.py b/src/streamlink/plugins/mediavitrina.py
--- a/src/streamlink/plugins/mediavitrina.py
+++ b/src/streamlink/plugins/mediavitrina.py
@@ -27,7 +27,7 @@
)\.ru/(?:live|online)""", re.VERBOSE))
@pluginmatcher(re.compile(r"https?://player\.mediavitrina\.ru/.+/player\.html"))
class MediaVitrina(Plugin):
- _re_url_json = re.compile(r"https://media\.mediavitrina\.ru/(?:proxy)?api/v3/\w+/playlist/[\w-]+_as_array\.json[^\"']+")
+ _re_url_json = re.compile(r"https://media\.mediavitrina\.ru/(?:proxy)?api/v3/[\w-]+/playlist/[\w-]+_as_array\.json[^\"']+")
def _get_streams(self):
self.session.http.headers.update({"Referer": self.url})
| diff --git a/tests/plugins/test_mediavitrina.py b/tests/plugins/test_mediavitrina.py
--- a/tests/plugins/test_mediavitrina.py
+++ b/tests/plugins/test_mediavitrina.py
@@ -34,6 +34,7 @@ class TestPluginCanHandleUrlMediaVitrina(PluginCanHandleUrl):
"https://player.mediavitrina.ru/tvc/tvc/moretv_web/player.html",
"https://player.mediavitrina.ru/tvzvezda/moretv_web/player.html",
"https://player.mediavitrina.ru/u_ott/u/moretv_web/player.html",
+ "https://player.mediavitrina.ru/gpm_tnt_v2/tnt/vitrinatv_web/player.html",
]
should_not_match = [
| plugins.mediavitrina: no playable streams found on player URLs
### Checklist
- [X] This is a plugin issue and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest stable release
### Description
Since january streamlink can't handle gazprom-media mediavitrina urls like:
https://player.mediavitrina.ru/gpm_tnt_v2/tnt/vitrinatv_web/player.html
https://player.mediavitrina.ru/gpm_friday_v2/friday/vitrinatv_web/player.html
https://player.mediavitrina.ru/tv3_v2/tv3/vitrinatv_web/player.html
The reason for that is beause mediavitrina can't open a required json file like
https://media.mediavitrina.ru/api/v3/gpm-tnt/playlist/tnt_as_array.json?application_id=&player_referer_hostname=vitrina.tv&config_checksum_sha256=&egress_version_id=1950111
what i know:
when i try to open this json file directly in browser it fails but when i specify a referer "https://player.mediavitrina.ru/" for media.mediavitrina.ru url using firefox extension it opens perfectly
so i think mediavitrina plugin does not send this referer requesting json from media.mediavitrina.ru URL, it sends referer only for player.mediavitrina.ru URLs
please fix this issue
P.S.:
it would be futureproof if this plugin just could handle https://media.mediavitrina.ru/api/v1/gpm-tnt/playlist/tnt_as_array.json URLs directly
### Debug log
```text
[cli][info] Found matching plugin mediavitrina for URL https://player.mediavitri
na.ru/gpm_tnt_v2/tnt/vitrinatv_web/player.html
error: No playable streams found on this URL: https://player.mediavitrina.ru/gpm
_tnt_v2/tnt/vitrinatv_web/player.html
```
| Post the full `--loglevel=debug` output, as requested by the plugin issue template.
sorry guys i was wrong on referer
i have found a solution: plugin just can't handle json URL address due to recently (in january) added "-" in "gpm-tnt"
so this line
_re_url_json = re.compile(r"https://media\.mediavitrina\.ru/(?:proxy)?api/v3/\w+/playlist/[\w-]+_as_array\.json[^\"']+")
should be replaced with this one
_re_url_json = re.compile(r"https://media\.mediavitrina\.ru/(?:proxy)?api/v3/[\w-]+/playlist/[\w-]+_as_array\.json[^\"']+")
it worked for me!!!
Submit a pull request | 2023-06-05T18:23:49 |
streamlink/streamlink | 5,390 | streamlink__streamlink-5390 | [
"5389"
] | 3fcf6d6c0903d55a263492570538933f4741ef06 | diff --git a/src/streamlink/plugins/showroom.py b/src/streamlink/plugins/showroom.py
--- a/src/streamlink/plugins/showroom.py
+++ b/src/streamlink/plugins/showroom.py
@@ -6,6 +6,7 @@
import logging
import re
+from urllib.parse import parse_qsl, urlparse
from streamlink.plugin import Plugin, pluginmatcher
from streamlink.plugin.api import validate
@@ -30,16 +31,18 @@ def _get_streams(self):
self.url,
schema=validate.Schema(
validate.parse_html(),
- validate.xml_xpath_string(".//script[contains(text(),'share_url:\"https:')][1]/text()"),
+ validate.xml_xpath_string(".//nav//a[contains(@href,'/room/profile?')]/@href"),
validate.none_or_all(
- re.compile(r"share_url:\"https:[^?]+?\?room_id=(?P<room_id>\d+)\""),
- validate.any(None, validate.get("room_id")),
+ validate.transform(lambda _url_profile: dict(parse_qsl(urlparse(_url_profile).query))),
+ validate.get("room_id"),
),
),
)
if not room_id:
return
+ log.debug(f"Room ID: {room_id}")
+
live_status, self.title = self.session.http.get(
"https://www.showroom-live.com/api/live/live_info",
params={
| plugins.showroom: streamlink recording a different showroom stream than the one specified
### Checklist
- [X] This is a plugin issue and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [x] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest stable release
### Description
Recently showroom.com has made some changes to their site.
When I try to use streamlink to record a showroom url that is offline, for eg. https://www.showroom-live.com/r/46_ikedateresa
> streamlink --loglevel debug https://www.showroom-live.com/r/46_ikedateresa best -o testing.ts`
the expected behavior is that it should return this:
> This stream is currently offline
error: No playable streams found on this URL: https://www.showroom-live.com/r/46_ikedateresa`
However, when I tried entering the above command 40-50 times in a row (tried both manually and using a .bat file), streamlink started recording a random different Showroom stream that is currently online. If I close streamlink and repeat the entire process again, it will record another random online stream.
This outcome only happened since a few days ago.
I have been using a bat script (since 2017) to get streamlink to keep repeating the same command every few seconds until the showroom stream turns online, so that I can record the stream when I am away. But now with the changes made, streamlink will be led to recording a different stream, so when the original stream that it is supposed to record begins, it won't record that anymore.
Bat script I use:
```bat
@echo off
::set _task=notepad.exe
set _task=streamlink
goto CHECK
:CHECK
REM Check whether the process is running
qprocess >tasklist_tmp.txt
type tasklist_tmp.txt | findstr /i %_task%
if %errorlevel% ==0 goto SLEEP
if %errorlevel% ==1 goto RUN
:RUN
set _suffix=.ts
set _command=streamlink --loglevel debug --stream-segment-threads 10 --stream-segment-timeout 15.0 --stream-segment-attempts 12 --hls-live-edge 2 --ringbuffer-size 900M --hls-playlist-reload-attempts 10 https://www.showroom-live.com/46_SHIHO_KATO best -o
::set _current_time=%date:~0,4%%date:~5,2%%date:~8,2%-%time:~0,2%%time:~3,2%%time:~6,2%
::set _service=%_command%%_current_time%%_suffix%
rem echo Get current date
for /f "tokens=1,2,3 delims=/- " %%a in ("%date%") do @set D=%%a%%b%%c
rem echo Get current time
for /f "tokens=1,2,3 delims=:." %%a in ("%time%") do @set T=%%a%%b%%c
rem echo If current hour less than 10£¬add 0 before hour
set T=%T: =0%
rem echo display current date and time
echo %D%%T%
set _service=%_command%L:\46_ikedateresa-%D%%T%%_suffix%
echo %_service%
::start c:\windows\system32\notepad.exe
start %_service%
echo The program started at %time%>>deamon.log
:SLEEP
REM sleep for 10 minutes
echo %time% The Program is Running,sleep for 5s...
echo Wscript.Sleep WScript.Arguments(0) >%tmp%\delay.vbs
cscript //b //nologo %tmp%\delay.vbs 3000
goto CHECK
```
### Debug log
```text
Expected result when recording a showroom stream that is yet to start:
L:\>streamlink --loglevel debug https://www.showroom-live.com/r/46_ikedateresa best -o testing1.ts
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.10.6
[cli][debug] Streamlink: 5.5.1
[cli][debug] Dependencies:
[cli][debug] certifi: 2022.6.15
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.1
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.16.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.28.2
[cli][debug] urllib3: 1.26.12
[cli][debug] websocket-client: 1.4.0
[cli][debug] Arguments:
[cli][debug] url=https://www.showroom-live.com/r/46_ikedateresa
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --output=testing1.ts
[cli][info] Found matching plugin showroom for URL https://www.showroom-live.com/r/46_ikedateresa
[plugins.showroom][info] This stream is currently offline
error: No playable streams found on this URL: https://www.showroom-live.com/r/46_ikedateresa
---------------------------------------------------------------------------------------------------
Unexpected result after 40-50 attempts: streamlink starts recording a different showroom stream (the stream that it is supposed to record have yet to start)
L:\>streamlink --loglevel debug https://www.showroom-live.com/r/46_ikedateresa best -o testing1.ts
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.10.6
[cli][debug] Streamlink: 5.5.1
[cli][debug] Dependencies:
[cli][debug] certifi: 2022.6.15
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.1
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.16.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.28.2
[cli][debug] urllib3: 1.26.12
[cli][debug] websocket-client: 1.4.0
[cli][debug] Arguments:
[cli][debug] url=https://www.showroom-live.com/r/46_ikedateresa
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --output=testing1.ts
[cli][info] Found matching plugin showroom for URL https://www.showroom-live.com/r/46_ikedateresa
[utils.l10n][debug] Language code: en_US
[cli][info] Available streams: 144p (worst), 360p, 720p (best)
[cli][info] Opening stream: 720p (hls)
[cli][info] Writing output to
L:\testing1.ts
[cli][debug] Checking file output
[stream.hls][debug] Reloading playlist
[cli][debug] Pre-buffering 8192 bytes
[stream.hls][debug] First Sequence: 32034; Last Sequence: 32038
[stream.hls][debug] Start offset: 0; Duration: None; Start Sequence: 32036; End Sequence: None
[stream.hls][debug] Adding segment 32036 to queue
[stream.hls][debug] Adding segment 32037 to queue
[stream.hls][debug] Adding segment 32038 to queue
[stream.hls][debug] Writing segment 32036 to output
[stream.hls][debug] Segment 32036 complete
[cli][debug] Writing stream to output
[download] Written 419.51 KiB to testing1.ts (0s) [stream.hls][debug] Writing segment 32037 to output
[stream.hls][debug] Segment 32037 complete
[download] Written 875.55 KiB to testing1.ts (0s) [stream.hls][debug] Writing segment 32038 to output
[stream.hls][debug] Segment 32038 complete
[stream.hls][debug] Reloading playlist
[download] Written 1.26 MiB to testing1.ts (1s) [stream.hls][debug] Adding segment 32039 to queue
[download] Written 1.26 MiB to testing1.ts (1s) [stream.hls][debug] Writing segment 32039 to output
[stream.hls][debug] Segment 32039 complete
[download] Written 1.65 MiB to testing1.ts (2s @ 672.65 KiB/s) [stream.hls][debug] Reloading playlist
[download] Written 1.65 MiB to testing1.ts (3s @ 611.75 KiB/s) [stream.hls][debug] Adding segment 32040 to queue
[download] Written 1.65 MiB to testing1.ts (3s @ 560.78 KiB/s) [stream.hls][debug] Writing segment 32040 to output
[stream.hls][debug] Segment 32040 complete
[download] Written 2.03 MiB to testing1.ts (4s @ 458.55 KiB/s) [stream.hls][debug] Reloading playlist
[stream.hls][debug] Adding segment 32041 to queue
```
| Here's the plugin code:
https://github.com/streamlink/streamlink/blob/5.5.1/src/streamlink/plugins/showroom.py
What you describe suggests that an incorrect room ID gets found when the channel is offline. The actual HLS stream URL is retrieved from their API which requires a specific room ID as one of the parameters, and it also checks for the online status first using the room ID.
The room ID currently gets extracted from some `share_url` object property in one of their embedded JS scripts, so this is probably not the right solution. | 2023-06-20T23:46:15 |
|
streamlink/streamlink | 5,396 | streamlink__streamlink-5396 | [
"5395"
] | 9c6dd568216da4e20be32e85413551a8cb2219e4 | diff --git a/src/streamlink_cli/argparser.py b/src/streamlink_cli/argparser.py
--- a/src/streamlink_cli/argparser.py
+++ b/src/streamlink_cli/argparser.py
@@ -1329,7 +1329,7 @@ def false(_): return False # noqa: E704
def setup_session_options(session: Streamlink, args: argparse.Namespace):
for arg, option, mapper in _ARGUMENT_TO_SESSIONOPTION:
value = getattr(args, arg)
- if value is not None:
+ if value:
if mapper is not None:
value = mapper(value)
session.set_option(option, value)
| diff --git a/tests/cli/test_argparser.py b/tests/cli/test_argparser.py
--- a/tests/cli/test_argparser.py
+++ b/tests/cli/test_argparser.py
@@ -59,23 +59,6 @@ def test_setup_session_options(parser: ArgumentParser, session: Streamlink, argv
assert session.get_option(option) == expected
[email protected](("default", "new", "expected"), [
- pytest.param(False, None, False, id="Default False, unset"),
- pytest.param(True, None, True, id="Default True, unset"),
- pytest.param(False, False, False, id="Default False, set to False"),
- pytest.param(False, True, True, id="Default False, set to True"),
- pytest.param(True, False, False, id="Default True, set to False"),
- pytest.param(True, True, True, id="Default True, set to True"),
-])
-def test_setup_session_options_override(monkeypatch: pytest.MonkeyPatch, session: Streamlink, default, new, expected):
- arg = "NON_EXISTING_ARGPARSER_ARGUMENT"
- key = "NON-EXISTING-SESSION-OPTION-KEY"
- monkeypatch.setattr("streamlink_cli.argparser._ARGUMENT_TO_SESSIONOPTION", [(arg, key, None)])
- session.set_option(key, default)
- setup_session_options(session, Namespace(**{arg: new}))
- assert session.get_option(key) == expected
-
-
def test_cli_main_setup_session_options(monkeypatch: pytest.MonkeyPatch, parser: ArgumentParser, session: Streamlink):
class StopTest(Exception):
pass
| cli.argparser: allow disabling session options
`streamlink_cli.argparser` should be able to set session options which default to `True` to `False`. Fix that by checking whether the argument dest on the argparse namespace is not `None` (aka set).
Currently, there are no session options which default to `True`.
----
Required for being able to add `--webbrowser=false` with respective default value of `True` on the Streamlink session.
| I did a mistake here. This unintentionally now always sets `--http-no-ssl-verify`, which is bad.
Please don't immediately merge PRs without a review. I sometimes make mistakes. | 2023-06-22T21:13:34 |
streamlink/streamlink | 5,408 | streamlink__streamlink-5408 | [
"5407",
"5407"
] | ee27aba93da61c822fff2ee7db4c581b0bfbfa33 | diff --git a/src/streamlink/plugins/nrk.py b/src/streamlink/plugins/nrk.py
--- a/src/streamlink/plugins/nrk.py
+++ b/src/streamlink/plugins/nrk.py
@@ -8,7 +8,6 @@
import logging
import re
-from urllib.parse import urljoin
from streamlink.plugin import Plugin, pluginmatcher
from streamlink.plugin.api import validate
@@ -23,83 +22,85 @@
r"https?://(?:tv|radio)\.nrk\.no/(program|direkte|serie|podkast)(?:/.+)?/([^/]+)",
))
class NRK(Plugin):
- _psapi_url = "https://psapi.nrk.no"
- # Program type to manifest type mapping
- _program_type_map = {
+ _URL_MANIFEST = "https://psapi.nrk.no/playback/manifest/{manifest_type}/{program_id}"
+ _URL_METADATA = "https://psapi.nrk.no/playback/metadata/{manifest_type}/{program_id}?eea-portability=true"
+
+ _MAP_MANIFEST_TYPE = {
"direkte": "channel",
"serie": "program",
"program": "program",
"podkast": "podcast",
}
- _program_id_re = re.compile(r'<meta property="nrk:program-id" content="([^"]+)"')
-
- _playable_schema = validate.Schema(validate.any(
- {
- "playable": {
- "assets": [{
- "url": validate.url(),
- "format": str,
- }],
- },
- "statistics": {
- validate.optional("luna"): validate.any(None, {
- "data": {
- "title": str,
- "category": validate.any(None, str),
+ def _get_metadata(self, manifest_type, program_id):
+ url_metadata = self._URL_METADATA.format(manifest_type=manifest_type, program_id=program_id)
+ non_playable = self.session.http.get(url_metadata, schema=validate.Schema(
+ validate.parse_json(),
+ {
+ validate.optional("nonPlayable"): validate.none_or_all(
+ {
+ "reason": str,
+ "endUserMessage": str,
},
- }),
+ validate.union_get("reason", "endUserMessage"),
+ ),
},
- },
- {
- "nonPlayable": {
- "reason": str,
- },
- },
- ))
+ validate.get("nonPlayable"),
+ ))
+ if non_playable:
+ reason, end_user_message = non_playable
+ log.error(f"Not playable: {reason} - {end_user_message or 'error'}")
+ return False
+
+ return True
+
+ def _update_program_id(self, program_id):
+ new_program_id = self.session.http.get(self.url, schema=validate.Schema(
+ validate.parse_html(),
+ validate.xml_xpath_string(".//meta[@property='nrk:program-id']/@content"),
+ ))
+ return new_program_id or program_id
+
+ def _get_assets(self, manifest_type, program_id):
+ return self.session.http.get(
+ self._URL_MANIFEST.format(manifest_type=manifest_type, program_id=program_id),
+ schema=validate.Schema(
+ validate.parse_json(),
+ {
+ "playable": {
+ "assets": [
+ validate.all(
+ {
+ "url": validate.url(),
+ "format": str,
+ },
+ validate.union_get("format", "url"),
+ ),
+ ],
+ },
+ },
+ validate.get(("playable", "assets")),
+ ),
+ )
def _get_streams(self):
- # Construct manifest URL for this program.
program_type, program_id = self.match.groups()
- manifest_type = self._program_type_map.get(program_type)
+ manifest_type = self._MAP_MANIFEST_TYPE.get(program_type)
if manifest_type is None:
log.error(f"Unknown program type '{program_type}'")
- return None
-
- # Fetch program_id.
- res = self.session.http.get(self.url)
- m = self._program_id_re.search(res.text)
- if m is not None:
- program_id = m.group(1)
- elif program_id is None:
- log.error("Could not extract program ID from URL")
- return None
-
- manifest_url = urljoin(self._psapi_url, f"playback/manifest/{manifest_type}/{program_id}")
-
- # Extract media URL.
- res = self.session.http.get(manifest_url)
- manifest = self.session.http.json(res, schema=self._playable_schema)
- if "nonPlayable" in manifest:
- reason = manifest["nonPlayable"]["reason"]
- log.error(f"Not playable ({reason})")
- return None
- self._set_metadata(manifest)
- asset = manifest["playable"]["assets"][0]
-
- # Some streams such as podcasts are not HLS but plain files.
- if asset["format"] == "HLS":
- return HLSStream.parse_variant_playlist(self.session, asset["url"])
- else:
- return [("live", HTTPStream(self.session, asset["url"]))]
-
- def _set_metadata(self, manifest):
- luna = manifest.get("statistics").get("luna")
- if not luna:
return
- data = luna["data"]
- self.category = data.get("category")
- self.title = data.get("title")
+
+ program_id = self._update_program_id(program_id)
+ if self._get_metadata(manifest_type, program_id) is False:
+ return
+
+ assets = self._get_assets(manifest_type, program_id)
+
+ # just return the first item
+ for stream_type, stream_url in assets:
+ if stream_type == "HLS":
+ return HLSStream.parse_variant_playlist(self.session, stream_url)
+ return [("live", HTTPStream(self.session, stream_url))]
__plugin__ = NRK
| plugins.nrk: error: Unable to validate JSON
### Checklist
- [X] This is a plugin issue and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest stable release
### Description
NRK appears to have removed `statistics` from the json at https://psapi.nrk.no/playback/metadata/channel/nrk1?eea-portability=true which breaks the plugin.
Extracting the m3u8 link from the browser and using it works.
### Debug log
```text
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.11.0
[cli][debug] Streamlink: 5.5.1
[cli][debug] Dependencies:
[cli][debug] certifi: 2022.9.24
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.2
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.18.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.29.0
[cli][debug] urllib3: 1.26.13
[cli][debug] websocket-client: 1.4.2
[cli][debug] Arguments:
[cli][debug] url=https://tv.nrk.no/direkte/nrk1
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --ffmpeg-ffmpeg=C:\Program Files (x86)\Streamlink\ffmpeg\ffmpeg.exe
[cli][info] Found matching plugin nrk for URL https://tv.nrk.no/direkte/nrk1
error: Unable to validate JSON: ValidationError(AnySchema):
ValidationError(dict):
Unable to validate value of key 'statistics'
Context(dict):
Unable to validate value of key 'luna'
Context(AnySchema):
ValidationError(equality):
<{'config': {'beacon': 'https://some-none-existing-alias...> does not equal None
ValidationError(dict):
Unable to validate value of key 'data'
Context(dict):
Key 'category' not found in <{'title': '', 'device': '', 'playerId': '', 'deliveryTy...>
ValidationError(dict):
Unable to validate value of key 'nonPlayable'
Context(type):
Type of None should be dict, but is NoneType
```
plugins.nrk: error: Unable to validate JSON
### Checklist
- [X] This is a plugin issue and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest stable release
### Description
NRK appears to have removed `statistics` from the json at https://psapi.nrk.no/playback/metadata/channel/nrk1?eea-portability=true which breaks the plugin.
Extracting the m3u8 link from the browser and using it works.
### Debug log
```text
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.11.0
[cli][debug] Streamlink: 5.5.1
[cli][debug] Dependencies:
[cli][debug] certifi: 2022.9.24
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.2
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.18.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.29.0
[cli][debug] urllib3: 1.26.13
[cli][debug] websocket-client: 1.4.2
[cli][debug] Arguments:
[cli][debug] url=https://tv.nrk.no/direkte/nrk1
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --ffmpeg-ffmpeg=C:\Program Files (x86)\Streamlink\ffmpeg\ffmpeg.exe
[cli][info] Found matching plugin nrk for URL https://tv.nrk.no/direkte/nrk1
error: Unable to validate JSON: ValidationError(AnySchema):
ValidationError(dict):
Unable to validate value of key 'statistics'
Context(dict):
Unable to validate value of key 'luna'
Context(AnySchema):
ValidationError(equality):
<{'config': {'beacon': 'https://some-none-existing-alias...> does not equal None
ValidationError(dict):
Unable to validate value of key 'data'
Context(dict):
Key 'category' not found in <{'title': '', 'device': '', 'playerId': '', 'deliveryTy...>
ValidationError(dict):
Unable to validate value of key 'nonPlayable'
Context(type):
Type of None should be dict, but is NoneType
```
| 2023-07-01T15:20:40 |
||
streamlink/streamlink | 5,414 | streamlink__streamlink-5414 | [
"5370"
] | 7bfaf395053a036e067419f68ebd64f160d1b4d4 | diff --git a/src/streamlink/plugins/twitch.py b/src/streamlink/plugins/twitch.py
--- a/src/streamlink/plugins/twitch.py
+++ b/src/streamlink/plugins/twitch.py
@@ -8,23 +8,27 @@
"""
import argparse
+import base64
import logging
import re
import sys
from datetime import datetime, timedelta
+from json import dumps as json_dumps
from random import random
-from typing import List, NamedTuple, Optional
+from typing import List, Mapping, NamedTuple, Optional, Tuple
from urllib.parse import urlparse
from streamlink.exceptions import NoStreamsError, PluginError
from streamlink.plugin import Plugin, pluginargument, pluginmatcher
from streamlink.plugin.api import validate
+from streamlink.session import Streamlink
from streamlink.stream.hls import HLSStream, HLSStreamReader, HLSStreamWorker, HLSStreamWriter
from streamlink.stream.hls_playlist import M3U8, ByteRange, DateRange, ExtInf, Key, M3U8Parser, Map, load as load_hls_playlist
from streamlink.stream.http import HTTPStream
from streamlink.utils.args import keyvalue
from streamlink.utils.parse import parse_json, parse_qsd
-from streamlink.utils.times import hours_minutes_seconds
+from streamlink.utils.random import CHOICES_ALPHA_NUM, random_token
+from streamlink.utils.times import fromtimestamp, hours_minutes_seconds
from streamlink.utils.url import update_qsd
@@ -406,7 +410,7 @@ def metadata_clips(self, clipname):
),
))
- def access_token(self, is_live, channel_or_vod):
+ def access_token(self, is_live, channel_or_vod, client_integrity: Optional[Tuple[str, str]] = None):
query = self._gql_persisted_query(
"PlaybackAccessToken",
"0828119ded1c13477966434e15800ff57ddacf13ba1911c129dc2200705b0712",
@@ -424,7 +428,11 @@ def access_token(self, is_live, channel_or_vod):
validate.union_get("signature", "value"),
)
- return self.call(query, acceptable_status=(200, 400, 401, 403), schema=validate.Schema(
+ headers = {}
+ if client_integrity:
+ headers["Device-Id"], headers["Client-Integrity"] = client_integrity
+
+ return self.call(query, acceptable_status=(200, 400, 401, 403), headers=headers, schema=validate.Schema(
validate.any(
validate.all(
{"errors": [{"message": str}]},
@@ -450,7 +458,7 @@ def access_token(self, is_live, channel_or_vod):
),
},
validate.get("data"),
- validate.transform(lambda data: ("token", *data)),
+ validate.transform(lambda data: ("token", *data) if data is not None else ("token", None, None)),
),
),
))
@@ -501,6 +509,117 @@ def stream_metadata(self, channel):
))
+class TwitchClientIntegrity:
+ URL_P_SCRIPT = "https://k.twitchcdn.net/149e9513-01fa-4fb0-aad4-566afd725d1b/2d206a39-8ed7-437e-a3be-862e0f06eea3/p.js"
+
+ # language=javascript
+ JS_INTEGRITY_TOKEN = """
+ // noinspection JSIgnoredPromiseFromCall
+ new Promise((resolve, reject) => {
+ function configureKPSDK() {
+ // noinspection JSUnresolvedVariable,JSUnresolvedFunction
+ window.KPSDK.configure([{
+ "protocol": "https:",
+ "method": "POST",
+ "domain": "gql.twitch.tv",
+ "path": "/integrity"
+ }]);
+ }
+
+ async function fetchIntegrity() {
+ // noinspection JSUnresolvedReference
+ const headers = Object.assign(HEADERS, {"x-device-id": "DEVICE_ID"});
+ // window.fetch gets overridden and the patched function needs to be used
+ const resp = await window.fetch("https://gql.twitch.tv/integrity", {
+ "headers": headers,
+ "body": null,
+ "method": "POST",
+ "mode": "cors",
+ "credentials": "omit"
+ });
+
+ if (resp.status !== 200) {
+ throw new Error(`Unexpected integrity response status code ${resp.status}`);
+ }
+
+ return JSON.stringify(await resp.json());
+ }
+
+ document.addEventListener("kpsdk-load", configureKPSDK, {once: true});
+ document.addEventListener("kpsdk-ready", () => fetchIntegrity().then(resolve, reject), {once: true});
+
+ const script = document.createElement("script");
+ script.addEventListener("error", reject);
+ script.src = "SCRIPT_SOURCE";
+ document.body.appendChild(script);
+ });
+ """
+
+ @classmethod
+ def acquire(
+ cls,
+ session: Streamlink,
+ channel: str,
+ headers: Mapping[str, str],
+ device_id: str,
+ ) -> Optional[Tuple[str, int]]:
+ from streamlink.webbrowser.cdp import CDPClient, CDPClientSession, devtools
+ from streamlink.webbrowser.exceptions import WebbrowserError
+
+ url = f"https://www.twitch.tv/{channel}"
+ js_get_integrity_token = cls.JS_INTEGRITY_TOKEN \
+ .replace("SCRIPT_SOURCE", cls.URL_P_SCRIPT) \
+ .replace("HEADERS", json_dumps(headers)) \
+ .replace("DEVICE_ID", device_id)
+ eval_timeout = session.get_option("webbrowser-timeout")
+
+ async def on_main(client_session: CDPClientSession, request: devtools.fetch.RequestPaused):
+ async with client_session.alter_request(request) as cm:
+ cm.body = "<!doctype html>"
+
+ async def acquire_client_integrity_token(client: CDPClient):
+ client_session: CDPClientSession
+ async with client.session() as client_session:
+ client_session.add_request_handler(on_main, url_pattern=url, on_request=True)
+ async with client_session.navigate(url) as frame_id:
+ await client_session.loaded(frame_id)
+ return await client_session.evaluate(js_get_integrity_token, timeout=eval_timeout)
+
+ try:
+ client_integrity: Optional[str] = CDPClient.launch(session, acquire_client_integrity_token)
+ except WebbrowserError as err:
+ log.error(f"{type(err).__name__}: {err}")
+ return None
+ if not client_integrity:
+ return None
+
+ token, expiration = parse_json(client_integrity, schema=validate.Schema(
+ {"token": str, "expiration": int},
+ validate.union_get("token", "expiration"),
+ ))
+ is_bad_bot = cls.decode_client_integrity_token(token, schema=validate.Schema(
+ {"is_bad_bot": str},
+ validate.get("is_bad_bot"),
+ validate.transform(lambda val: val.lower() != "false"),
+ ))
+ log.info(f"Is bad bot? {is_bad_bot}")
+ if is_bad_bot:
+ return None
+
+ return token, expiration / 1000
+
+ @staticmethod
+ def decode_client_integrity_token(data: str, schema: Optional[validate.Schema] = None):
+ if not data.startswith("v4.public."):
+ raise PluginError("Invalid client-integrity token format")
+ token = data[len("v4.public."):].replace("-", "+").replace("_", "/")
+ token += "=" * ((4 - (len(token) % 4)) % 4)
+ token = base64.b64decode(token.encode())[:-64].decode()
+ log.debug(f"Client-Integrity token: {token}")
+
+ return parse_json(token, exception=PluginError, schema=schema)
+
+
@pluginmatcher(re.compile(r"""
https?://(?:(?P<subdomain>[\w-]+)\.)?twitch\.tv/
(?:
@@ -573,7 +692,14 @@ def stream_metadata(self, channel):
Can be repeated to add multiple parameters.
""",
)
+@pluginargument(
+ "purge-client-integrity",
+ action="store_true",
+ help="Purge cached Twitch client-integrity token and acquire a new one.",
+)
class Twitch(Plugin):
+ _CACHE_KEY_CLIENT_INTEGRITY = "client-integrity"
+
@classmethod
def stream_weight(cls, stream):
if stream == "source":
@@ -638,17 +764,52 @@ def _get_metadata(self):
except (PluginError, TypeError):
pass
+ def _client_integrity_token(self, channel: str) -> Optional[Tuple[str, str]]:
+ if self.options.get("purge-client-integrity"):
+ log.info("Removing cached client-integrity token...")
+ self.cache.set(self._CACHE_KEY_CLIENT_INTEGRITY, None, 0)
+
+ client_integrity = self.cache.get(self._CACHE_KEY_CLIENT_INTEGRITY)
+ if client_integrity and isinstance(client_integrity, list) and len(client_integrity) == 2:
+ log.info("Using cached client-integrity token")
+ device_id, token = client_integrity
+ else:
+ log.info("Acquiring new client-integrity token...")
+ device_id = random_token(32, CHOICES_ALPHA_NUM)
+ client_integrity = TwitchClientIntegrity.acquire(
+ self.session,
+ channel,
+ self.api.headers,
+ device_id,
+ )
+ if not client_integrity:
+ log.warning("No client-integrity token acquired")
+ return None
+
+ token, expiration = client_integrity
+ self.cache.set(self._CACHE_KEY_CLIENT_INTEGRITY, [device_id, token], expires_at=fromtimestamp(expiration))
+
+ return device_id, token
+
def _access_token(self, is_live, channel_or_vod):
- try:
- response, *data = self.api.access_token(is_live, channel_or_vod)
+ # try without a client-integrity token first (the web player did the same on 2023-05-31)
+ response, *data = self.api.access_token(is_live, channel_or_vod)
+
+ # try again with a client-integrity token if the API response was erroneous
+ if response != "token":
+ client_integrity = self._client_integrity_token(channel_or_vod) if is_live else None
+ response, *data = self.api.access_token(is_live, channel_or_vod, client_integrity)
+
+ # unknown API response error: abort
if response != "token":
error, message = data
- log.error(f"{error or 'Error'}: {message or 'Unknown error'}")
- raise PluginError
- sig, token = data
- except (PluginError, TypeError):
- raise NoStreamsError # noqa: B904
+ raise PluginError(f"{error or 'Error'}: {message or 'Unknown error'}")
+
+ # access token response was empty: stream is offline or channel doesn't exist
+ if response == "token" and data[0] is None:
+ raise NoStreamsError
+ sig, token = data
try:
restricted_bitrates = self.api.parse_token(token)
except PluginError:
diff --git a/src/streamlink/utils/random.py b/src/streamlink/utils/random.py
new file mode 100644
--- /dev/null
+++ b/src/streamlink/utils/random.py
@@ -0,0 +1,14 @@
+from random import choice
+
+
+CHOICES_NUM = "0123456789"
+CHOICES_ALPHA_LOWER = "abcdefghijklmnopqrstuvwxyz"
+CHOICES_ALPHA_UPPER = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"
+CHOICES_ALPHA = f"{CHOICES_ALPHA_LOWER}{CHOICES_ALPHA_UPPER}"
+CHOICES_ALPHA_NUM = f"{CHOICES_NUM}{CHOICES_ALPHA}"
+CHOICES_HEX_LOWER = f"{CHOICES_NUM}{CHOICES_ALPHA_LOWER[:6]}"
+CHOICES_HEX_UPPER = f"{CHOICES_NUM}{CHOICES_ALPHA_UPPER[:6]}"
+
+
+def random_token(length: int = 32, choices: str = CHOICES_ALPHA_NUM) -> str:
+ return "".join(choice(choices) for _ in range(length))
| diff --git a/tests/plugins/test_twitch.py b/tests/plugins/test_twitch.py
--- a/tests/plugins/test_twitch.py
+++ b/tests/plugins/test_twitch.py
@@ -6,7 +6,7 @@
import requests_mock as rm
from streamlink import Streamlink
-from streamlink.exceptions import NoStreamsError
+from streamlink.exceptions import NoStreamsError, PluginError
from streamlink.options import Options
from streamlink.plugins.twitch import Twitch, TwitchAPI, TwitchHLSStream, TwitchHLSStreamReader, TwitchHLSStreamWriter
from tests.mixins.stream_hls import EventedHLSStreamWriter, Playlist, Segment as _Segment, Tag, TestMixinStreamHLS
@@ -396,6 +396,11 @@ def test_hls_low_latency_no_ads_reload_time(self):
class TestTwitchAPIAccessToken:
+ @pytest.fixture(autouse=True)
+ def _client_integrity_token(self, monkeypatch: pytest.MonkeyPatch):
+ mock_client_integrity_token = Mock(return_value=("device-id", "client-integrity-token"))
+ monkeypatch.setattr(Twitch, "_client_integrity_token", mock_client_integrity_token)
+
@pytest.fixture()
def plugin(self, request: pytest.FixtureRequest):
session = Streamlink()
@@ -409,7 +414,7 @@ def plugin(self, request: pytest.FixtureRequest):
def mock(self, request: pytest.FixtureRequest, requests_mock: rm.Mocker):
mock = requests_mock.post("https://gql.twitch.tv/gql", **getattr(request, "param", {"json": {}}))
yield mock
- assert mock.call_count == 1
+ assert mock.call_count > 0
payload = mock.last_request.json() # type: ignore[union-attr]
assert tuple(sorted(payload.keys())) == ("extensions", "operationName", "variables")
assert payload.get("operationName") == "PlaybackAccessToken"
@@ -480,7 +485,7 @@ def _assert_vod(self, mock: rm.Mocker):
),
], indirect=["plugin"])
def test_plugin_options(self, plugin: Twitch, mock: rm.Mocker, exp_headers: dict, exp_variables: dict):
- with pytest.raises(NoStreamsError):
+ with pytest.raises(PluginError):
plugin._access_token(True, "channelname")
requestheaders = dict(mock.last_request._request.headers) # type: ignore[union-attr]
for header in plugin.session.http.headers.keys():
@@ -505,6 +510,7 @@ def test_live_success(self, plugin: Twitch, mock: rm.Mocker):
def test_live_failure(self, plugin: Twitch, mock: rm.Mocker):
with pytest.raises(NoStreamsError):
plugin._access_token(True, "channelname")
+ assert len(mock.request_history) == 1, "Only gets the access token once when the channel is offline"
@pytest.mark.usefixtures("_assert_vod")
@pytest.mark.parametrize("mock", [{
@@ -521,6 +527,7 @@ def test_vod_success(self, plugin: Twitch, mock: rm.Mocker):
def test_vod_failure(self, plugin: Twitch, mock: rm.Mocker):
with pytest.raises(NoStreamsError):
plugin._access_token(False, "vodid")
+ assert len(mock.request_history) == 1, "Only gets the access token once when the VOD doesn't exist"
@pytest.mark.usefixtures("_assert_live")
@pytest.mark.parametrize(("plugin", "mock"), [
@@ -532,13 +539,50 @@ def test_vod_failure(self, plugin: Twitch, mock: rm.Mocker):
},
),
], indirect=True)
- def test_auth_failure(self, caplog: pytest.LogCaptureFixture, plugin: Twitch, mock: rm.Mocker):
- with pytest.raises(NoStreamsError):
+ def test_auth_failure(self, plugin: Twitch, mock: rm.Mocker):
+ with pytest.raises(PluginError, match="^Unauthorized: The \"Authorization\" token is invalid\\.$"):
plugin._access_token(True, "channelname")
- assert mock.last_request._request.headers["Authorization"] == "OAuth invalid-token" # type: ignore[union-attr]
- assert [(record.levelname, record.module, record.message) for record in caplog.records] == [
- ("error", "twitch", "Unauthorized: The \"Authorization\" token is invalid."),
- ]
+ assert len(mock.request_history) == 2, "Always tries again on error, with integrity-token on second attempt"
+
+ headers: dict = mock.request_history[0]._request.headers
+ assert headers["Authorization"] == "OAuth invalid-token"
+ assert "Device-Id" not in headers
+ assert "Client-Integrity" not in headers
+
+ headers = mock.request_history[1]._request.headers
+ assert headers["Authorization"] == "OAuth invalid-token"
+ assert headers["Device-Id"] == "device-id"
+ assert headers["Client-Integrity"] == "client-integrity-token"
+
+ @pytest.mark.usefixtures("_assert_live")
+ @pytest.mark.parametrize(("plugin", "mock"), [
+ (
+ [("api-header", [("Authorization", "OAuth invalid-token")])],
+ {"response_list": [
+ {
+ "status_code": 401,
+ "json": {"errors": [{"message": "failed integrity check"}]},
+ },
+ {
+ "json": {"data": {"streamPlaybackAccessToken": {"value": '{"channel":"foo"}', "signature": "sig"}}},
+ },
+ ]},
+ ),
+ ], indirect=True)
+ def test_failed_integrity_check(self, plugin: Twitch, mock: rm.Mocker):
+ data = plugin._access_token(True, "channelname")
+ assert data == ("sig", '{"channel":"foo"}', [])
+ assert len(mock.request_history) == 2, "Always tries again on error, with integrity-token on second attempt"
+
+ headers: dict = mock.request_history[0]._request.headers
+ assert headers["Authorization"] == "OAuth invalid-token"
+ assert "Device-Id" not in headers
+ assert "Client-Integrity" not in headers
+
+ headers = mock.request_history[1]._request.headers
+ assert headers["Authorization"] == "OAuth invalid-token"
+ assert headers["Device-Id"] == "device-id"
+ assert headers["Client-Integrity"] == "client-integrity-token"
class TestTwitchMetadata:
diff --git a/tests/utils/test_random.py b/tests/utils/test_random.py
new file mode 100644
--- /dev/null
+++ b/tests/utils/test_random.py
@@ -0,0 +1,69 @@
+from collections import defaultdict
+from itertools import count
+from typing import Dict, Iterator, Sequence
+
+import pytest
+
+from streamlink.utils.random import (
+ CHOICES_ALPHA,
+ CHOICES_ALPHA_LOWER,
+ CHOICES_ALPHA_NUM,
+ CHOICES_ALPHA_UPPER,
+ CHOICES_HEX_LOWER,
+ CHOICES_HEX_UPPER,
+ CHOICES_NUM,
+ random_token,
+)
+
+
[email protected]()
+def _iterated_choice(monkeypatch: pytest.MonkeyPatch):
+ choices: Dict[Sequence, Iterator[int]] = defaultdict(lambda: count())
+
+ def fake_choice(seq):
+ return seq[next(choices[seq]) % len(seq)]
+
+ monkeypatch.setattr("streamlink.utils.random.choice", fake_choice)
+
+
[email protected]("_iterated_choice")
[email protected](("args", "expected"), [
+ (
+ dict(),
+ "0123456789abcdefghijklmnopqrstuv",
+ ),
+ (
+ dict(length=62),
+ "0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ",
+ ),
+ (
+ dict(length=20, choices=CHOICES_NUM),
+ "01234567890123456789",
+ ),
+ (
+ dict(length=52, choices=CHOICES_ALPHA_LOWER),
+ "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyz",
+ ),
+ (
+ dict(length=52, choices=CHOICES_ALPHA_UPPER),
+ "ABCDEFGHIJKLMNOPQRSTUVWXYZABCDEFGHIJKLMNOPQRSTUVWXYZ",
+ ),
+ (
+ dict(length=104, choices=CHOICES_ALPHA),
+ "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ",
+ ),
+ (
+ dict(length=100, choices=CHOICES_ALPHA_NUM),
+ "0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789abcdefghijklmnopqrstuvwxyzAB",
+ ),
+ (
+ dict(length=32, choices=CHOICES_HEX_LOWER),
+ "0123456789abcdef0123456789abcdef",
+ ),
+ (
+ dict(length=32, choices=CHOICES_HEX_UPPER),
+ "0123456789ABCDEF0123456789ABCDEF",
+ ),
+])
+def test_random_token(args, expected):
+ assert random_token(**args) == expected
| plugins.twitch: no playable streams on this url 2023-05-31
### Checklist
- [X] This is a plugin issue and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest build from the master branch
### Description
twitch streams are no longer loading for me, giving the message `No playable streams found on this url`. Tested on both latest release and master.
In addition I tried disabling twitch-specific options in the debug log like `twitch-disable-ads` and `twitch-low-latency` but the same error happens regardless.
### Debug log
```text
[cli][debug] OS: Linux-6.3.2-arch1-1-x86_64-with-glibc2.37
[cli][debug] Python: 3.11.3
[cli][debug] Streamlink: 5.5.1+41.g9e05e321
[cli][debug] Dependencies:
[cli][debug] certifi: 2022.12.7
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.2
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.17
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.28.2
[cli][debug] urllib3: 1.26.15
[cli][debug] websocket-client: 1.5.1
[cli][debug] importlib-metadata: 5.0.0
[cli][debug] Arguments:
[cli][debug] url=https://twitch.tv/gamesdonequick
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --player=mpv
[cli][debug] --default-stream=['best']
[cli][debug] --twitch-disable-ads=True
[cli][debug] --twitch-low-latency=True
[cli][info] Found matching plugin twitch for URL https://twitch.tv/gamesdonequick
[plugins.twitch][debug] Getting live HLS streams for gamesdonequick
error: No playable streams found on this URL: https://twitch.tv/gamesdonequick
```
| Twitch killed all third party tools and adblockers by this recent change, this is the response of the GQL (where the playlist is fetched) of anyone using streamlink
```json
{
"data": {
"streamPlaybackAccessToken": null
},
"errors": [
{
"message": "failed integrity check",
"path": [
"streamPlaybackAccessToken"
]
}
],
"extensions": {
"challenge": {
"type": "integrity"
},
"durationMilliseconds": 1,
"operationName": "PlaybackAccessToken",
"requestID": "01H1SYB01BD45P11TQ7CMFT3G6"
}
}
```
As you can see they added some sort of integrity check (`"message": "failed integrity check"`), i don't really know if it can be bypassed.
*I just wanted to see forsen and streamlink never opened* :(
**Edit**: Even if you are subscribed to a channel and you have configured the `--twitch-api-header=Authorization=OAuth <token>` argument, you will not be able to open the stream.
Before we get too many comments here I'm going to ask that people only add a comment if they have useful and relevant data. There is no need for "me as well" or "I'm also running in to this" comments and those will be removed to keep the thread clean. I will pin this issue to try and avoid duplicate issues being created. Any duplicate issues will be closed and marked as a duplicate.
----
edit by @bastimeyer
# Please don't turn this into a help thread. If you want to discuss anything off-topic, then please use the Streamlink Matrix/Gitter channel
https://matrix.to/#/#streamlink_streamlink:gitter.im
Otherwise, we'll have to lock this thread, which would be annoying because this issue is still very much unsolved.
----
**See https://github.com/streamlink/streamlink/issues/5370#issuecomment-1572576728 for a temporary solution** (Twitch API Client-ID of the Nintendo Switch)
Looks like the `PlaybackAccessToken` GQL query requires the `Client-Integrity` HTTP header.
I can see two requests being made by my web browser, where one request fails with the same GQL error response and another one with that header succeeds. I have no idea yet where the header value is coming from, but its format is
```
v4.public.SOME-LONG-BASE64-VALUE
```
I had just gone to bed...
Minimum set of headers that seem to be checked as of now are `Authorization` (oauth token), `Client-Integrity` (expires every 16 hours based on `https://gql.twitch.tv/integrity`
response), `X-Device-Id` (unsure of expiry)
More information on the integrity token, it appears to be a jwt token with IP, user id, device id, and some sort of bot detection field
https://github.com/mauricew/twitch-graphql-api#integrity
edit: X-Device-Id needs to match the device id in Client-Integrity
not sure if related, but firefox also gets black screens on all twitch streams (not vods) as of today if you have `resistFingerprinting` in enabled
I'm also half in bed, so sorry for the "not that precise answer", but maybe it can give you some info.
```
curl 'https://gql.twitch.tv/integrity' -X POST -H 'Client-Id: xxxx'
```
Returns a token, but it is shorter than the full token. Full token is returned by
```
curl 'https://gql.twitch.tv/integrity' -X POST -H 'Client-Id: xxx' -H 'X-Device-Id: xxx' -H 'Client-Request-Id: xxx'
```
I do not know if the shorted token would work. I'll try to figure out what those `-Id` needs to be.
The `X-Device-Id` is the id set by the `unique_id` cookie on the initial html document response.
The `Client-Id` is the id of the client, that is hardcoded in the plugin <https://github.com/streamlink/streamlink/blob/9e05e321b013f8966fd932405f2e6b367159bb24/src/streamlink/plugins/twitch.py#L254>
Not sure about request id.
Without X-Device-Id, the integrity token has `"is_bad_bot": "true"` set, which blocks it from getting the playlist
edit: i have yet to figure out the right combination to get `is_bad_bot: false`
This is from the deobfuscated JS code
```js
return "https://gql.twitch.tv/integrity",
n = {
"Client-Id": this.config.authSettings.clientID,
"X-Device-Id": this.session.deviceID,
"Client-Request-Id": e,
"Client-Session-Id": this.session.appSessionID,
"Client-Version": this.config.buildID
},
this.authToken && (n.Authorization = "OAuth ".concat(this.authToken)),
[4, fetch("https://gql.twitch.tv/integrity", {
headers: n,
method: "POST"
})];
```
- `Client-Id` is the regular one
- `X-Device-Id` is the `unique_id` cookie set when accessing `https://twitch.tv/` for the first time
- `Client-Request-Id` is a randomized 32 bytes long alphanumerical string (random for each request)
- `Client-Session-Id` is a randomized 16 bytes long hex string (stays for the entire session)
- `Client-Version` is a uuid4 string, can be read from embedded JS on `https://twitch.tv/` or from the JSON response from `https://static.twitchcdn.net/config/manifest.json?v=1`
No success so far though. I'm getting an integrity token, but it's not valid when trying to get an access token
related https://github.com/Kappador/twitch-integrity/issues/1
this may have to do with kasada as well, they gave up on it because they got in an arms race with kasada's bot detection
> not sure if related, but firefox also gets black screens on all twitch streams (not vods) as of today if you have `resistFingerprinting` in enabled
I have "privacy.resistFingerprinting = True" in 'about:config', and I can load twitch streams just fine. I cannot confirm this black screen behavior.

Even replaying an api request to `https://gql.twitch.tv/integrity` (copy as curl with all headers) gets me a token with `is_bad_bot: true` set.
edit: they're base64 encoded jwt tokens that's where the attributes come from
Some more details here, regarding the kasada bot detection. https://github.com/yt-dlp/yt-dlp/issues/7183#issuecomment-1571109121
> Prior research on this integrity check, including knowledge of why `privacy.resistFingerprinting` is implicated: https://digipres.club/@moralrecordings/109496127240969369
>
> GitHub repo that appears to mention the same fields, and points fingers at which anti-automation framework is involved: https://github.com/unicorn-aio/kpsdk
In order to get a valid integrity token, you'll also need the `x-kpsdk-cd`, `x-kpsdk-ct`, `x-kpsdk-v` headers ("kasada bot detection").
Here's the relevant JS section
```js
n.prototype.loadKPSDK = function() {
return (0,
i.__awaiter)(this, void 0, Promise, (function() {
var e, n = this;
return (0,
i.__generator)(this, (function(t) {
return this.loadPromise || (e = !1,
this.loadPromise = new Promise((function(t, r) {
var a = performance.now()
, o = function(e) {
return n.trackKPSDKLatency(a, e)
};
document.addEventListener(f.Load, (function() {
var r;
if (!e) {
o(g.Loaded);
var a = (0,
i.__spreadArray)([], n.endpointsToProtectFromConstructor, !0);
if ((0,
N.t7)("auth_kpsdk_load")) {
var s = new URL(_e.config.passportBaseURL);
a.push({
protocol: s.protocol,
method: "POST",
domain: s.host,
path: "/integrity"
})
}
null === (r = window.KPSDK) || void 0 === r || r.configure(a),
a.length || t()
}
}
)),
document.addEventListener(f.Ready, (function() {
e || (o(g.Ready),
t())
}
));
var s = n.dynamicSettings.get("kpsdk_timeout", 15e3);
setTimeout((function() {
n.isKPSDKReady() || (o(g.Timeout),
e = !0,
r())
}
), s);
var l = document.createElement("script");
l.src = "https://k.twitchcdn.net/149e9513-01fa-4fb0-aad4-566afd725d1b/2d206a39-8ed7-437e-a3be-862e0f06eea3/p.js",
document.body.appendChild(l)
}
))),
[2, this.loadPromise]
}
))
}
))
}
```
Which loads the following JS script (this will probably change a lot with lots of updates)
`https://k.twitchcdn.net/149e9513-01fa-4fb0-aad4-566afd725d1b/2d206a39-8ed7-437e-a3be-862e0f06eea3/p.js`
and which adds the `window.KPSDK` object.
And if you take a look at this, you'll see that a JS runtime is required.
# R I P
@bastimeyer Would this work? `https://github.com/PiotrDabkowski/Js2Py`
The language is not an issue here. These anti-bot frameworks utilize subtle differences in browser JavaScript engines to verify whether it's a real browser. They probably also use TLS fingerprinting on the server side to check whether the request came from a real browser (not checked on Twitch, although this would be fairly trivial to bypass).
I can confirm I can fetch a valid integrity token using a headless Chrome with a few modifications, e.g., screen size.
(Adding here to not clutter the discussion)
> Assuming python gets access to a browser, could it lift the credentials from the browser after the stream is opened there to hand it off to streamlink?
> Couldn't we pass integrity check through chromium and then send stream URL to player?
If you have access to a browser, you can just get a m3u8 stream link. The whole purpose of these projects is to eliminate the need for a browser.
> Would this work?
No, because of ES6 (you can't just transpile it to ES5) and there's also no support for the DOM (I can see DOM nodes and DOM event stuff). This is meant to be executed by web browsers.
Have a look with "proper" formatting and synax highlighting:
https://gist.github.com/bastimeyer/d3bd77fbb6afc87460b2985393614caa
That's very likely it for the Twitch plugin and also for my Twitch GUI app...
I'm going to bed now...
----
> TLS fingerprinting
That isn't something which could be solved here anyway, if they actually do that. That'd have to be resolved by urllib3 or by httpx.
> I can confirm I can fetch a valid integrity token using a headless Chrome with a few modifications, e.g., screen size.
Couldn't we pass integrity check through chromium and then send stream URL to player?
@thinkpad4 usually, the issue is not the javascript engine (you could use quickjs from https://bellard.org ) but the web engine.
Gotcha @sylware
I'd be surprised if this was useful, but I still have a streamlink session open to Twitch. It should remain up for the next several days (fingers crossed). I imagine it'd be tough connecting to the running Python process--but I believe it's doable if necessary.
What is the intention behind this? Keeping the bots away? That won't work. The code is obfuscated, but you can always reverse engineer it because it's also public, otherwise the concept of the service doesn't work. All they are doing is buying a little time. DOMReady and the pseudo encryption can be implemented as well.
A ton of obfuscation won't save Twitch on that front. Now they've burned through a lot of money again just to get this monstrosity done. The timing is also peculiar, right before Blizzard releases D4. In the end, streamers are just losing viewers. Is that the goal? Fewer viewers?
@thinkpad4 they want to force you to use their big tech web engines (blink/webkit) and will do anything to "break" any alternative, usually the msft way: adding complexity and size until nobody can replicate, goodely enough, the dynamic behavior... which would be what is fingerprinted.
@git42leech they want to sell ads, so they thought removing bots would restore the trust of the advertisers, which is wrong or malicious: the first one the advertisers would not trust is twitch itself as they can forge their metrics much more easily than sellers of viewers, for their own benefits or their friends'.
The only reliable metrics for the advertisers on internet are their actual sales.
Yep, this is plain wrong, and malicious as it tries to trick advertisers into giving more trust in their own metrics.
Or they want to reduce the cost of their bandwidth by removing bots traffic.
Let's try to stay on topic with the issue at hand being how to work with the integrity token rather than clog up the thread with comments bashing Twitch.
I don't understand about these things but if it helps in any way I'll post the request made by the Twitch app for Android. I will remove some values as they may be sensitive to my account.
```
POST /gql HTTP/1.1
X-APOLLO-OPERATION-ID: [REDACTED]
X-APOLLO-OPERATION-NAME: StreamAccessTokenQuery
Accept: multipart/mixed; deferSpec=20220824, application/json
X-APOLLO-CAN-BE-BATCHED: false
X-APOLLO-OPERATION-ID: [REDACTED]
X-APOLLO-OPERATION-NAME: StreamAccessTokenQuery
Accept: multipart/mixed; deferSpec=20220824, application/json
Content-Type: application/json
Content-Length: 268
Host: [gql.twitch.tv](http://gql.twitch.tv/)
Connection: Keep-Alive
Accept-Encoding: gzip
Client-ID: [REDACTED]
Authorization: OAuth [REDACTED]
Accept-Language: pt-br
X-Device-ID: [REDACTED]
Api-Consumer-Type: mobile; Android/1502010
X-App-Version: 15.2.1
Client-Session-Id: [REDACTED]
User-Agent: Dalvik/2.1.0 (Linux; U; Android 8.1.0; SM-J710MN Build/M1AJQ) [tv.twitch.android.app/15.2.1/1502010](http://tv.twitch.android.app/15.2.1/1502010)
{"operationName":"StreamAccessTokenQuery","variables":{"channelName":"olkabone","params":{"platform":"android","playerType":"mobile_player"}},"extensions":{"persistedQuery":{"version":1,"sha256Hash":"4aeb12b75899a00ed245a3bd272047407f60a15f473caaa8a8e64719b6f6a8d5"}}}
```
FWIW, Android client still works https://github.com/crackededed/Xtra so you can look how they extract streams.
@jebibot true. But for the purposes of @bastimeyer's GUI project, a userscript or something that reduces browser use to once/16hr by spitting out 5000 base64 chars encoding all this week's secret handshakes would be better than nothing. For the moment though, could you share code snippets describing how you coerced valid tokens out of a headless chrome? I have my own wrapper script I'd like to repair.
> FWIW, Android client still works https://github.com/crackededed/Xtra so you can look how they extract streams.
I noticed this earlier. However their ability to proxy the m3u8 request is broken or luminous-ttv's public server is down.
>luminous-ttv's public server is down
luminous-ttv is broken due to the changes (source: am its developer), as well as TTV-LOL v1 and similar services
Hello, everyone.
In fact, there is a solution...
first, Selenium or similar tools at the front end to receive the following header information: `Authorization`, `Client-Id`, `Client-Integrity`, `X-Device-Id`.
second, with this information, you can construct and execute the CLI command as shown below.
```
streamlink "twitch.tv/STREAMER_NAME" "best" -o "output.ts" \
--twitch-api-header="Authorization=OAuth [REDACTED]" \
--twitch-api-header="Client-Id=[REDACTED]" \
--twitch-api-header="Client-Integrity=[REDACTED]" \
--twitch-api-header="X-Device-Id=[REDACTED]"
```
Please note that the headers obtained using this method are valid for **only 16 hours**.
Keep this in mind and understand that this is not a fundamental solution.
anyway,,, I hope you have a great day! thanks :)
>
> And if you take a look at this, you'll see that a JS runtime is required.
> # R I P
Do you think it is worth it to try to write a tool, using bun for example, that would run this JS and get the integrity header? I do not know exactly how strong this bot detection is.
Potential relevant issue regarding to kdsdk
https://orionfeedback.org/d/4601-twitch-stopped-to-recgonise-the-browser-independent-of-configuration/21
https://github.com/WebKit/WebKit/pull/10760
> Hello, everyone.
>
> In fact, there is a solution...
>
> first, Selenium or similar tools at the front end to receive the following header information: `Authorization`, `Client-Id`, `Client-Integrity`, `X-Device-Id`. second, with this information, you can construct and execute the CLI command as shown below.
>
> ```
> streamlink "twitch.tv/STREAMER_NAME" "best" -o "output.ts" \
> --twitch-api-header="Authorization=OAuth [REDACTED]" \
> --twitch-api-header="Client-Id=[REDACTED]" \
> --twitch-api-header="Client-Integrity=[REDACTED]" \
> --twitch-api-header="X-Device-Id=[REDACTED]"
> ```
>
> Please note that the headers obtained using this method are valid for **only 16 hours**. Keep this in mind and understand that this is not a fundamental solution.
>
> anyway,,, I hope you have a great day! thanks :)
I can confirm this works, just open any stream while logged into your account in any browser that supports "Network Inspector" like tools, copy the necessary headers out, and I'm getting the actual stream retrieved.
The "valid only every 16 hours" thing is definitely going to be obnoxious to deal with, but I'm able to get the neccesary headers in Firefox that's loaded down with NoScript and Β΅Block Origin still operating over the entire page.
I hate to ask but where do I find this information exactly in the browser console tools ? i looked everywhere i possibly can like in "storage inspector" where it lists all the websites - player.twitch.tv, gql.twitch.tv, etc and i cant find ANY entries that say "client id", client integrity, or X-device-id
i found API token and Auth Token, and all my stuff like my username and and so on, but those other 3 entries are no where to be found whatsoever. And i dont know how exactly where to go to get those values or info. or what to type in the "console" box. I tried the one mentioned in the streamlink documents that mentions how to get your Auth token, and that works. But that's it.
I apoligize for my newbie-ness, i dont know anything about javascript and other programming languages on how to find this information.
https://i.ibb.co/p1Q6sKJ/twitch-stuff.png
where do i go to find those missing values ? :( (x device, client id, and client integrity) i already tried the front twitch page and they dont show up there either.
@kujakiller go to network, search GQL, click a request with POST, look at the headers part, copy from there

# Please don't turn this into a help thread. If you want to discuss anything off-topic, then please use the Streamlink Matrix/Gitter channel
https://matrix.to/#/#streamlink_streamlink:gitter.im
Otherwise, we'll have to lock this thread, which would be annoying because this issue is still very much unsolved.
----
> In fact, there is a solution...
Yes, as mentioned earlier, but I don't know how realistic it is to use Selenium for that and ship it with Streamlink on all platforms.
Fetching the access token like that btw is exactly the same as getting the HLS URL directly.
I'll have to leave the house now and will be home later. I already have a local branch which sets all those headers, as mentioned earlier, but the integrity token obviously requires external tools.
@KynikossDragonn
Hmm... I don't want to discourage you, but the truth is, this is an incomplete solution.
Among these headers, the Client-Integrity has the characteristic of changing for each public IP address.
So, for example, if you copy and execute the header value on an AWS EC2 instance, it won't work.
Therefore, tools like Selenium are necessary, and these web tools have a larger scope than the problem itself, making it difficult to call them a proper solution.
It would be better to consider it as simply a temporary measure or emergency treatment.
I would prefer not to provide specific instructions on how to use this solution.
> Hello, everyone.
>
> In fact, there is a solution...
>
> first, Selenium or similar tools at the front end to receive the following header information: `Authorization`, `Client-Id`, `Client-Integrity`, `X-Device-Id`. second, with this information, you can construct and execute the CLI command as shown below.
>
> ```
> streamlink "twitch.tv/STREAMER_NAME" "best" -o "output.ts" \
> --twitch-api-header="Authorization=OAuth [REDACTED]" \
> --twitch-api-header="Client-Id=[REDACTED]" \
> --twitch-api-header="Client-Integrity=[REDACTED]" \
> --twitch-api-header="X-Device-Id=[REDACTED]"
> ```
>
> Please note that the headers obtained using this method are valid for **only 16 hours**. Keep this in mind and understand that this is not a fundamental solution.
>
> anyway,,, I hope you have a great day! thanks :)
But you cannot have a valid `Client-Integrity` <-> `X-Device-Id` when you load the Twitch using headless browsers, because they are considered as "bad-bot"s in the integrity check and you'll receive an error when you pass them to `/gql` endpoint.
> > Hello, everyone.
> > In fact, there is a solution...
> > first, Selenium or similar tools at the front end to receive the following header information: `Authorization`, `Client-Id`, `Client-Integrity`, `X-Device-Id`. second, with this information, you can construct and execute the CLI command as shown below.
> > ```
> > streamlink "twitch.tv/STREAMER_NAME" "best" -o "output.ts" \
> > --twitch-api-header="Authorization=OAuth [REDACTED]" \
> > --twitch-api-header="Client-Id=[REDACTED]" \
> > --twitch-api-header="Client-Integrity=[REDACTED]" \
> > --twitch-api-header="X-Device-Id=[REDACTED]"
> > ```
> >
> >
> >
> >
> >
> >
> >
> >
> >
> >
> >
> > Please note that the headers obtained using this method are valid for **only 16 hours**. Keep this in mind and understand that this is not a fundamental solution.
> > anyway,,, I hope you have a great day! thanks :)
>
> But you cannot have a valid `Client-Integrity` <-> `X-Device-Id` when you load the Twitch using headless browsers, because they are considered as "bad-bot"s in the integrity check and you'll receive an error when you pass them to `/gql` endpoint.
Not really, there's a tons of solutions to deep fake real browser from headless, see Undetected ChromeDriver.
But this should not be our final solution because it's too heavy.
Python does support faking real web browser by using tls-client library: https://github.com/FlorianREGAZ/Python-Tls-Client
Which is a choice for streamlink, but we should be able to find a better solution without using any dependency.
Pepega Clap
```js
// Hardcoded
const challengeType = {
difficulty: 10,
platformInputs: "tp-v2-input",
subchallengeCount: 2
};
// https://passport.twitch.tv/<uuid-v4>/<uuid_v4>/fp?x-kpsdk-v=j-0.0.0
// Then get KPSDK.message
const rawMessage = 'KPSDK:DONE:XXX::true:2:999';
const challengeHeader = {
FIELD_NAME_CHALLENGE_DATA: "kpsdkCd",
KPSDK_HEADER_CHALLENGE_DATA: "x-kpsdk-cd",
// Other data as I don't know where to put them
KPSDK_HEADER_CLIENT_TOKEN: "x-kpsdk-ct",
KPSDK_HEADER_VERSION: "x-kpsdk-v"
};
let serverConfig = {}; // Initialized by parseMessageConfig
function parseMessageConfig(msg) {
if ("string" == typeof msg && "KPSDK:DONE" === msg.substring(0x0, 0xa)) {
let splitMsg = msg.split(":").map(decodeURIComponent)
, encodedClientToken = splitMsg[2]
, cryptoChallengeEnabled = splitMsg[4]
, serverTime = splitMsg[6];
return {
encodedClientToken: encodedClientToken.length > 0 ? encodedClientToken : void 0x0,
cryptoChallengeEnabled: "true" === cryptoChallengeEnabled,
serverTime: Number(serverTime)
};
}
}
const challengeModuleV2Data = {
time: 0, // _0x33781b
serverTime: 0, // _0x5b0222
deltaArr: []
};
const challengeModuleV2 = {
headerNames: ["x-kpsdk-cd"],
formFieldNames: challengeHeader.FIELD_NAME_CHALLENGE_DATA
};
let globalOffset = 0;
function calcGLobalOffset(time, serverTime) {
let delta = time - serverTime; // "time" is Date.now() I think
challengeModuleV2Data.time = time;
challengeModuleV2Data.serverTime = serverTime;
challengeModuleV2Data.deltaArr.push(delta);
if (challengeModuleV2Data.deltaArr.length === 1)
globalOffset = delta;
else
globalOffset = Math.round(challengeModuleV2Data.deltaArr.length / challengeModuleV2Data.deltaArr.reduce(function(accumulator, currentValue) {
return accumulator + 0x1 / currentValue;
}, 0));
}
function makeId() {
for (var str = '', i = 0x0; i < 0x20; i += 0x1)
str += ('0123456789abcdef')[Math.floor(0x10 * Math.random())];
return str;
}
function sha256(str) {
// Should be any generic sha256 function (https://emn178.github.io/online-tools/sha256.html)
// Sample implementation taken from https://github.com/brillout/forge-sha256/blob/master/build/forge-sha256.js
return window.forge_sha256(str);
}
function calcDifficulty(hash) {
return 0x10000000000000 / (Number('0x'.concat(hash.slice(0x0, 0xd))) + 0x1);
}
function calcHash(challengeTypeObj, delta, currId) {
// Random value for testing I guess
// "tp-v2-input, 1685607313121, 075695085525b25727553b31e7403f38" (everything within quotes) =>
// 912b55b6376f7b286ef298b8028d67176fd1a3987aa95c1a951c40e3fd73e6b1
for (
var currHash = sha256("".concat(challengeTypeObj["platformInputs"], ", ", delta, ", ", currId)),
actualDiff = challengeTypeObj["difficulty"] / challengeTypeObj["subchallengeCount"],
answerArr = [],
j = 0; j < challengeTypeObj["subchallengeCount"]; j += 1
) {
for (var i = 1; ; ) {
var tempHash = sha256("".concat(i, ", ", currHash));
if (calcDifficulty(tempHash) >= actualDiff) {
answerArr.push(i),
currHash = tempHash;
break;
}
i += 1;
}
}
return {
answers: answerArr,
finalHash: currHash
};
}
function solveChallengeRaw(challengeTypeObj, serverOffset, testId = undefined) { // testId for testing with random id
var time = Date.now()
, beforeTime = performance.now()
, delta = time - serverOffset
, currId = testId === undefined ? makeId() : testId
, resHash = calcHash(challengeTypeObj, delta, currId)
, afterTime = performance.now()
, duration = Math.round(1000 * (afterTime - beforeTime)) / 1000;
return {
workTime: delta,
id: currId,
answers: resHash.answers,
duration: duration,
d: serverOffset,
st: 0x0,
rst: 0x0
};
}
function solveChallenge(challengeType) {
let res = solveChallengeRaw(challengeType, globalOffset);
res.st = challengeModuleV2Data.serverTime;
res.rst = challengeModuleV2Data.time;
return res;
}
function makeSolution(msg, headers, challengeType) {
/*
"headers" should be something like this:
{
"Client-Id": "xxx",
"X-Device-Id": "xxx",
"Client-Request-Id": "xxx",
"Client-Session-Id": "xxx",
"Client-Version": "xxx"
}
//*/
let tempData = parseMessageConfig(msg);
tempData.solvedChallenge = solveChallenge(challengeType);
return {
interceptData: tempData,
init: {
"headers": headers,
"method": "POST"
}
}
}
function makeFeatureFlag() {
return {
featureFlags: {
"interception.version-header": true,
},
dynamicConfig: {
frontend: {
collectErrors: true,
collectTimings: true
}
}
};
}
function makeHeadersChallenge(solveSolution) {
// "solveSolution" are from solveChallenge(...)
return [challengeHeader.KPSDK_HEADER_CHALLENGE_DATA, JSON.stringify(solveSolution)];
}
function makeHeadersClient(solveData) {
return [challengeHeader.KPSDK_HEADER_CLIENT_TOKEN, solveData.encodedClientToken];
}
function makeGqlHeader(solution) {
// "solution" are from makeSolution(...)
return [
makeHeadersChallenge(solution['solvedChallenge']),
makeHeadersClient(solution.interceptData),
[challengeHeader.KPSDK_HEADER_VERSION, 'j-0.0.0']
];
}
```
Translated line by line from the obfuscated code. If anyone wants to test if headers are valid I guess.
Back to watching GDQ π
If anyone has the chance to clarify how that java script is used I'd greatly appreciate it. Else I'll just wait until a solution is implemented into a streamlink build.
vaft script on https://github.com/pixeltris/TwitchAdSolutions seems work now
> vaft script on https://github.com/pixeltris/TwitchAdSolutions seems work now
After taking a look, if I understand it correctly, I think it doesn't solve the challenge, it seems to add a hook to the browser requests, and get the `Client-Integrity` out of the browser. So this doesn't help us.
The code @Trung0246 posted seems fairly simple to re-implement in python. But I wonder if there is a "gotcha" that would make it hard to run outside a browser.
Maybe we can try first with a node js app, with the js code, and if we can get a working integrity header, we can rewrite it in python.
Something to watch out for would be other forms of browser fingerprinting we don't know about yet, and as mentioned much earlier in the thread from prior art on this work, that this ends up getting in an arms race with kasada.
> The code @Trung0246 posted seems fairly simple to re-implement in python.
This code is unfortunately not 100% complete, but it's a great help so far. I've been trying to implement the relevant KPSDK stuff in Python for the past two and a half hours or so, but there's still some stuff missing. Transpiling the obfuscated code by hand is really tedious and it already makes me want to write a BabelJS plugin for replacing all these stupid symbol lookups.
And yes, what's been posted here doesn't touch any DOM stuff, so a re-implementation in Python would be possible if this is indeed all that's needed for getting the integrity token request headers right.
All of this however comes down to the question of how often Twitch will make changes to the KPSDK. They are not using a third-party library like this for no reason. It's another game of cat and mouse, and it's an annoying one where figuring out changes is really hard. There's also the question when the hardcoded UUID4 strings on the "passport" message request will change. Currently those two seem hardcoded:
`149e9513-01fa-4fb0-aad4-566afd725d1b` and `2d206a39-8ed7-437e-a3be-862e0f06eea3`
The Selenium approach is also far from ideal and I'm not even sure if it's actually viable.
For now though I think trying to re-implement the `x-kpsdk-cd`, `x-kpsdk-ct` and `x-kpsdk-v` header values makes more sense.
Hm looks like there's some prior art, does these help? Just suddenly remembered I read somewhere about this instead of doing by hands like yesterday:
https://www.humansecurity.com/tech-engineering-blog/defeating-javascript-obfuscation
https://steakenthusiast.github.io/2022/05/21/Deobfuscating-Javascript-via-AST-An-Introduction-to-Babel/
Meanwhile I'll attempt to translate all relevant part, btw which part are still incomplete @bastimeyer ? Currently in the channel chat. Also somebody posted a Mastodon thread on some guys doing almost exact same thing like mine (I think in this issue, reposted here for bump): https://digipres.club/@moralrecordings/109496127240969369
Potential alternate approach: https://github.com/rdavydov/Twitch-Channel-Points-Miner-v2/issues/94#issuecomment-1360683876
> Looks like the `PlaybackAccessToken` GQL query requires the `Client-Integrity` HTTP header. I can see two requests being made by my web browser, where one request fails with the same GQL error response and another one with that header succeeds. I have no idea yet where the header value is coming from, but its format is
>
> ```
> v4.public.SOME-LONG-BASE64-VALUE
> ```
It looks like Paseto v4
https://github.com/paseto-standard/paseto-spec/blob/master/docs/Rationale-V3-V4.md
It's likely issued by the server.
I would not recommend solving this by reverse engineering the JS challenge because the challenge is trivial to mutate indefinitely & perpetually. This is an arms race that Kasada will always win.
The other strategy, making the scrapers indistinguishable from humans browsing the web, is a bit tricky because the selenium-based `undetected-chromedriver` is too smelly of a dependency.
This could also be achieved by spawning a local server and pointing the user's browsers at it to grab the token. This workflow is standard today for apps implementing Single Sing-On, like the Hashicorp Vault.
The default system browser can be opened, or `--twitch-browser` setting can be added.
Sequence:
1. Start a server thread, bound to loopback on a random port. E.g. `http.server`
2. Start the browser in stand-alone mode. E.g. `chrome --new-window --app="https://localhost:port" --start-maximized --disable-extensions --disable-plugins`
3. Complete the Twitch challenge
4. Tare down the browser and server process
> > > Hello, everyone.
> > > In fact, there is a solution...
> > > first, Selenium or similar tools at the front end to receive the following header information: `Authorization`, `Client-Id`, `Client-Integrity`, `X-Device-Id`. second, with this information, you can construct and execute the CLI command as shown below.
> > > ```
> > > streamlink "twitch.tv/STREAMER_NAME" "best" -o "output.ts" \
> > > --twitch-api-header="Authorization=OAuth [REDACTED]" \
> > > --twitch-api-header="Client-Id=[REDACTED]" \
> > > --twitch-api-header="Client-Integrity=[REDACTED]" \
> > > --twitch-api-header="X-Device-Id=[REDACTED]"
> > > ```
I was able to get a stream playing with the windows-build but not with the linux build (both latest build)
on linux in only get :
streamlink: error: argument --twitch-api-header: invalid keyvalue value: ''
the client-id stays the same across devices i noticed
you can grab all the id`s and tokens via the dev-tools from chrome by filtering the network tab for "gql" and looking at the request headers
The passport/gql `/fp` endpoints seem to respond differently depending on your cookies, without proper `KP_UIDz`/`ga__12_abel` cookies it will respond with another obfuscated javascript blob instead of `KPSDK.message`. Those cookies seem to come from POSTing some encrypted blob to the `/tl` endpoint (probably the output of the `/fp` javascript). It seems like this process is only done once, and then subsequent calls to `/fp` just return the token directly (I guess the `KP_UIDz` cookie indicates some kind of proof-of-work to the server so you only have to do it once). There's definitely quite a few more layers to this than the above de-obfuscated javascript alludes to.
> Potential alternate approach: [rdavydov/Twitch-Channel-Points-Miner-v2#94 (comment)](https://github.com/rdavydov/Twitch-Channel-Points-Miner-v2/issues/94#issuecomment-1360683876)
It seems that Client-ID they use is actually still completely unrestricted, specifying `--twitch-api-header Client-ID=ue6666qo983tsx6so1t0vnawi233wa` fixes it (seems to be the Client-ID from the Nintendo Switch?).
I can imagine this being another cat and mouse game though like with the old ad bypass headers.
> Hello, everyone.
>
> In fact, there is a solution...
>
> first, Selenium or similar tools at the front end to receive the following header information: `Authorization`, `Client-Id`, `Client-Integrity`, `X-Device-Id`. second, with this information, you can construct and execute the CLI command as shown below.
>
> ```
> streamlink "twitch.tv/STREAMER_NAME" "best" -o "output.ts" \
> --twitch-api-header="Authorization=OAuth [REDACTED]" \
> --twitch-api-header="Client-Id=[REDACTED]" \
> --twitch-api-header="Client-Integrity=[REDACTED]" \
> --twitch-api-header="X-Device-Id=[REDACTED]"
> ```
>
> Please note that the headers obtained using this method are valid for **only 16 hours**. Keep this in mind and understand that this is not a fundamental solution.
>
> anyway,,, I hope you have a great day! thanks :)
Will the stream process end once the keys expire? Or are these needed only for the initial opening of the stream?
This is a real stick in the mud for someone who had this whole VOD backup process automated >.<
> > Potential alternate approach: [rdavydov/Twitch-Channel-Points-Miner-v2#94 (comment)](https://github.com/rdavydov/Twitch-Channel-Points-Miner-v2/issues/94#issuecomment-1360683876)
>
> It seems that Client-ID they use is actually still completely unrestricted, specifying `--twitch-api-header Client-ID=ue6666qo983tsx6so1t0vnawi233wa` fixes it (seems to be the Client-ID from the Nintendo Switch?).
Awesome, I tested it and indeed simply using the flag `--twitch-api-header Client-ID=ue6666qo983tsx6so1t0vnawi233wa` completely fixes streamlink and makes it work again. That's an easy fix! π
> This is a real stick in the mud for someone who had this whole VOD backup process automated >.<
VODs aren't affected as far as I can tell (for the moment at least).
I previously had that as an `http-header` in my config.twitch file, but I had not considered making it a `twitch-api-header` too; the corresponding line would be
twitch-api-header=Client-ID=ue6666qo983tsx6so1t0vnawi233wa
and for good measure, go ahead and add this line too:
http-header=Client-ID=ue6666qo983tsx6so1t0vnawi233wa
That's a decent alternative to specifying command-line flags.
So to sum it up, there are hardcoded client ids for non-browser devices like tv, switch, etc that are not subject to the integrity check?
> > This is a real stick in the mud for someone who had this whole VOD backup process automated >.<
>
> VODs aren't affected as far as I can tell (for the moment at least).
Sorry for my improper wording but I record the streams to get an unmuted copy of the VOD for video editing
> So to sum it up, there are hardcoded client ids for non-browser devices like tv, switch, etc that are not subject to the integrity check?
I can indeed confirm that my Android-TV who is not logged into twitch plays any stream i tested also an chrome incognito tab does play any stream!
Well, I'm glad we have a workaround for now. I tested it on my recording script and it works fine!
> So to sum it up, there are hardcoded client ids for non-browser devices like tv, switch, etc that are not subject to the integrity check?
Probably a matter of time before it, too, is subject to the integrity check. Not fond of the idea of using it, but probably will use it for the time being.
> Probably a matter of time before it, too, is subject to the integrity check. Not fond of the idea of using it, but probably will use it for the time being.
likely depends on the app in question but it may not have a browser to easily do that, or twitch rotates the client ids, but that's speculation into the future for now. at least for now we have a workaround that currently works while we figure out the integrity check situation.
> does not work on my end..
Because you're using an outdated version or you're setting the CLI arguments wrong. See the install and CLI docs. It's not hard.
Just a quick reminder, don't turn this into a help thread. I will hide a couple of posts now, because it's derailing a bit. If you're having any problems setting the API headers, then ask on the Matrix/Gitter channel, as posted at the very top. Hope you understand that. We want to keep this thread at least a bit clean.
>just a quick reminder, don't turn this into a help thread. I will hide a couple of posts now, because it's derailing a bit. If you're having any problems setting the API headers, then ask on the Matrix/Gitter channel, as posted at the very top. Hope you understand that we want to keep this thread at least a bit clean.
Sorry my bad.. I deleted my postings that went off-topic
I can confirm that doing `streamlink --twitch-api-header Client-ID=ue6666qo983tsx6so1t0vnawi233wa https://www.twitch.tv/esl_csgo best` in the terminal has restored streaming functionality. I've put `--twitch-api-header Client-ID=ue6666qo983tsx6so1t0vnawi233wa` in Streamlink Twitch GUI's Settings -> Streaming -> Custom parameters and that restored its functionality as well. I am on Mac.
@Trung0246 Sorry about the incompleteness comment earlier. I have a tidied-up Python implementation here now which should set the correct headers. However, the passport.twitch.tv request results in a 429 response because it's missing cookies from what I can tell when making the same request with curl. This is another stupid obstacle :/
----
@DavidO2023
> I would not recommend solving this by reverse engineering the JS challenge because the challenge is trivial to mutate indefinitely & perpetually. This is an arms race that Kasada will always win.
Yes, you are right, and I think everyone else is aware of this too. As a short-term goal however this is easier to implement.
The Selenium approach shouldn't be too bad, but it would probably require more user configuration. I don't see it problematic having it as a dependency. The benefit here is that this can be run headless in various web browsers. The only question here is whether a working integrity token can be extracted.
An implementation of a local web server bridge is bad IMO, because it's really inconvenient having to manually navigate with your web browser to each stream in order to make the Twitch plugin work. Or when launching the web browser automatically, this would restrict it to Chromium-based browsers only via the `--app` argument in order to have it isolated and in order to be able to terminate the process again. These bridges are mainly intended for authentication purposes, but for something like opening streams, this is way too inconvenient and annoying. Not to mention that this breaks the CLI workflow.
If they go further with fingerprinting, it might be a good candidate for a browser extension. Loading twitch and grabbing whatever is necessary within a real browser.
It looks like you can't use both OAuth token and use Client-ID bypass like mentioned above at the same time..
> It looks like you can't use both OAuth token and use Client-ID bypass like mentioned above at the same time..
I'm able to do it when using the values pulled from firefox. At least with unsubscribed streams. None of the streamers I'm subscribed to have come on yet though. I'm on Linux.
@bs85 I guess it is time to start to code a python headless web engine, or give up and become a big tech web engine plugin+app (and since I have been using streamlink/yt-dlp to avoid those big tech web engines, that would be the end of this road for me).
Apologies if off-topic. This crude javascript might help anyone trying to get tokens from their browser, proof of concept you can extract tokens from dev tools console
```
const { fetch: originalFetch } = window;
window.fetch = async (...args) => {
let [resource, config ] = args;
if (
config.method === 'POST' &&
typeof config.headers['Authorization'] === 'string' &&
typeof config.headers['Client-Id'] === 'string' &&
typeof config.headers['Client-Integrity'] === 'string' &&
typeof config.headers['X-Device-Id'] === 'string'
) {
const {Authorization, 'Client-Id': ClientId, 'Client-Integrity': ClientIntegrity, 'X-Device-Id': DeviceID} = config.headers;
const cmd = `streamlink "${window.location.hostname}${window.location.pathname}" "best" \
--twitch-api-header="Authorization=${Authorization}" \
--twitch-api-header="Client-Id=${ClientId}" \
--twitch-api-header="Client-Integrity=${ClientIntegrity}" \
--twitch-api-header="X-Device-Id=${DeviceID}"`;
console.log(cmd);
}
const response = await originalFetch(resource, config);
return response;
};
```
> So to sum it up, there are hardcoded client ids for non-browser devices like tv, switch, etc that are not subject to the integrity check?
Let's hope the nintendo switch will never get a browser engine and we should be fine for some time.
> It looks like you can't use both OAuth token and use Client-ID bypass like mentioned above at the same time..
This works for me, I have twitch turbo and it loads all channel I tried without ads.
My config file:
```
default-stream=best
player=mpv
twitch-low-latency
twitch-api-header=Authorization=OAuth REDACTED
twitch-api-header=Client-Id=ue6666qo983tsx6so1t0vnawi233wa
```
With release `5.5.1`
Taking a hint from the game console client ID method, I tested Android and iOS user agents on desktop Firefox with an add-on and privacy hardened config to see what would happen. Turns out I was able to load live streams on the mobile site regardless of user agent, so it's likely that the problematic check hasn't been added to the mobile site (at least yet). Leveraging how they handle mobile devices is the easiest general route in the short term, but I don't know if it's viable in the long term
Definitely using `Client-Id` that does not require additional changes would be easiest short-term fix and monitor what is the behavior. Depending on Twitch's software team and their release cycles it might take some time until those mobile/embedded clients are updated and it is not clear what would be the verification on those. In general DESKTOP WEB client id is often used as first development target where they also test how certain things works.
> This works for me, I have twitch turbo and it loads all channel I tried without ads.
Thanks. Got it working
Streamlink/Twitch is working for me again suddenly? I do not have Twitch Turbo.
I use streamlink/twitch as a library from a python program. Didn't try anything to fix the error and in this moment started to work again.
They must of rolled back the kasada implementation as others are pointing out. I assume they're going to patch whatever holes in it that they can think of. I do not have the ```Client-ID``` header set in the streamlink launch options and I can open streams just fine now.
I can confirm they must have rolled it back since I am using only `--twitch-api-header=Authentication=OAuth <REDACTED>` as the header option and it works.
can confirm that `--twitch-api-header Client-ID=ue6666qo983tsx6so1t0vnawi233wa` works in fooling twitch into thinking streamlink is actually a Nintendo Switch.
Just wanted to note that, given the outage duration of almost exactly 24 hours, I believe this is a "planned deprecation warning" similar to those they've done in the past. When they deprecated the v5 "Kraken" API, they first did a 1 hour outage, followed by a 24 hour outage, followed by permanent. I feel like this is what they're doing here as well. Expect a permanent ~~outage of the current system~~ roll-forward of whatever they did in either 1 week or 1 month.
> > So to sum it up, there are hardcoded client ids for non-browser devices like tv, switch, etc that are not subject to the integrity check?
>
> Let's hope the nintendo switch will never get a browser engine and we should be fine for some time.
To be pedantic, the Switch does have a browser engine, but it only loads for captive portals; still, it would be inconvenient if Twitch required Switch-users to set up their own Wi-Fi access points as captive portals that point to Twitch (akin to how some efforts at jailbreaking involve the same sort of shenanigans, using a specially crafted access point to load an arbitrary Web page).
> To be pedantic, the Switch does have a browser engine, but it only loads for captive portals; still, it would be inconvenient if Twitch required Switch-users to set up their own Wi-Fi access points as captive portals that point to Twitch (akin to how some efforts at jailbreaking involve the same sort of shenanigans, using a specially crafted access point to load an arbitrary Web page).
the client id posted here is for Android TV anyway and not Switch. though there's probably Switch id that also works the same way
Let me give you a recap and an update on the current state about Twitch and plans for the plugin. I've already posted some of this on the Matrix/Gitter channel over the past days, but I'm going to repeat this here again, since more people will read this.
When trying to get an access token, Twitch's web player sets the `Client-Integrity` header. This was made a requirement last week, but it's currently disabled again, as you all know.
Some of it seems to be slightly different now or at least inconsistent, but when the requirement was set, the `Client-Integrity` header value was caluculated in these steps:
1. The web player loads `p.js` with lots of obfuscated JS code that requires a JS runtime.
2. `passport.twitch.tv/.../fp` gets requested and if no cookies from (3) are sent, it results in a 429 HTTP status code and another obfuscated JS script. This script contains string-encoded bytecode (~250 KiB) for a custom JS virtual machine that is then run, which is really complicated. I stepped through some of it where it interprets individual bytecode instructions and reads data from the bytecode, and I found a couple of things, like a block-encryption algorithm with a 128-bit key and 64-bit IV and lots of object+property names that are all related to browser-fingerprinting.
3. Once that script succeeds, it requests `passport.twitch.tv/.../tl` with a large binary `POST` body and the response to this are the `KP_UIDz` and `ga__12_abel` cookies which are probably valid for a longer time (didn't check).
4. `passport.twitch.tv/.../fp` gets requested again and with the right cookies it now returns status code 200 with a `KPSDK.message` string.
5. That message string then gets interpreted by `p.js` (1) where it calculates the `x-kpsdk-cd`, `x-kpsdk-ct` and `x-kpsdk-v` headers that are then set on the `gql.twitch.tv/integrity` GQL API endpoint.
6. That API response then gives us the `Client-Integrity` header value which we need to set on the GQL API query for getting a stream access token. When base64-decoding parts of the client-integrity token, it gives us a JSON object with the `is_bad_bot` key. If its value is `"true"`, then no access token gets returned.
Their web player currently still does the proof-of-work challenge (2) and sets all those headers (5), so we can still check when we're considered a "bad bot" (5), even though the `Client-Integrity` header is currently not a requirement.
----
All of this means that we'll have to use an actual web browser and use automation tools like Selenium (or others) for integrating the data extraction into Streamlink. The problem however becomes how well we can integrate this without causing too many setup/configuration/usability issues. Packaging is another problem in terms of Python dependencies, especially on Linux distros where dependencies are not bundled.
Selenium uses the webdriver interface of each supported browser. Over the past couple of days I've been working on a generic webdriver API in Streamlink that plugins could use and these were the initial problems:
1. The W3C "webdriver" protocol spec can't be used, because we need bi-directional communication with the web browser, because we need to intercept network requests.
2. The W3C "bidi webdriver" protocol spec is a work-in-progress and not implemented in Selenium yet.
3. The only alternative for bi-directional communication is the Chrome Devtools Protocol, which is limited to Chromium-based browsers and it's only partially supported by Firefox. For CDP we don't actually need Selenium and it's only useful as a lib for launching the browser and doing the async CDP communication (using `python-trio`, which is nice).
Then there are issues in regards to running browsers in headless mode (browser running "invisibly" and without requiring a desktop environment):
1. Chromium does have a "new" headless mode since release 112 (current one from a couple of days ago is 114), but it requires a new launch argument.
2. Safari still doesn't have a headless mode.
----
Now to my experimentation so far (not going to write down all the details):
Unfortunately, Twitch's browser fingerprinting and bot detection is really tough. It always detects when a browser is run and controlled via the webdriver, because the browser is run in a slightly different environment in this case. Running in headless mode also can be detected.
Anti-fingerprinting is of course nothing new for various browser-automation projects (Selenium, Puppeteer, Playwright, Cypress, etc.) and there are JS libs which try to reset the environment and try to make various fingerprint detection tools fail. For example, this one here, which bundles a collection of know bot-detection evasion scripts:
https://github.com/berstend/puppeteer-extra/tree/master/packages/extract-stealth-evasions
https://github.com/berstend/puppeteer-extra/tree/master/packages/puppeteer-extra-plugin-stealth/evasions
This unfortunately doesn't work on Twitch.
There's also the following service, which lots of sites implement. This might be unrelated to Twitch, but it's another thing that can be used to test whether a JS script thinks we're a bot.
https://fingerprint.com/products/bot-detection/
So with the webdriver implementation being ruled out, this means that Selenium won't work because that's how it interacts with the web browsers. Since I was already working with the Chrome Devtools Protocol (CDP) anyway, as the other protocols can't be used, Selenium was already unnecessary. We can launch the browser on our own if we restrict the whole thing to Chromium-based browsers anyway.
If you're not using the webdriver, Chromium can be controlled via the CDP by launching it with the `--remote-debugging-port=...` argument and setting an empty `--user-data-dir` (and some other optional OS/env-compatibility and initialization arguments). This is how some of the other browser-automation tools (still) do it, and that's also how I've implemented the test communication-bridge in Streamlink Twitch GUI years ago. All you need is a websocket connection for issuing CDP commands and listening to events. There are of course libs available with high-level APIs for that.
----
Long story short, at least in my tests so far, if you launch Chromium on your own with the remote debugging port and if you then connect to the CDP session and open Twitch's embedded player with a running stream, it'll perform the proof-of-work challenge and **it will set a `Client-Integrity` token which is NOT considered a "bad bot" as long as you DON'T run in headless mode**.
Headless mode still gets detected, which is a shame. This means is that if we want to run headlessly, we need to find out how to circumvent Twitch's bot detection and implement/add different/more scripts for fooling it.
As an alternative to the headless mode, launching the browser with a minimized window should work without causing too much disturbance. That however means that a desktop environment is required. I have no idea if an X virtual framebuffer works on Linux or if it also gets detected because some browser features are unavailable (if it can even run). In that sense, Twitch at least had achieved a proper bot-prevention while massively annoying regular users.
----
Final comments about a potential implementation in the Twitch plugin:
Since we've already figured out how to interpret the `KPSDK.message` format in order to set the headers on the `/integrity` endpoint (at least I think we do) in order to get the `Client-Integrity` header value, we could make launching the web browser only a requirement when we don't have the cookies from (2) or when they have expired. Once retrieved, Streamlink could cache them, so we don't have to launch the web browser next time. Whether that'll work depends on the correctness and stability of the `KPSDK.message` Python-implementation. But that's just a thought for an efficiency improvement once we have a working implementation.
Would having a browser extension be possible? Would it be able to support more browsers? And would it allow us to just use your normal browser and session?
Hm I think we could have users to open a link a html file (served by a very minimal webserver by streamlink) with a iframe of the `/fp` exactly like how it supposed to be within the twitch html source.
A sample log with that in mind could works like this:
```
[cli][info] Found matching plugin twitch for URL https://twitch.tv/gamesdonequick
[cli][info] Waiting for http://192.168.1.135:4545 to be opened in a browser due to missing cookies...
[cli][info] User opened the link and cookies are successfully retrieved.
[cli][info] Available streams: audio_only, 160p (worst), 360p, 480p, 720p60, 1080p60 (best)
[cli][info] Opening stream: 1080p60 (hls)
[cli][info] Starting player: "C:\Program Files\VideoLAN\VLC\vlc.exe"
```
The benefit of this method is we don't have to automate everything and still allow any browsers to be used (including while running streamlink in headless env like a VPS or a server which don't have an actual browser)
> Would having a browser extension be possible? Would it be able to support more browsers? And would it allow us to just use your normal browser and session?
Client-Integrity extraction from web browser should be the easiest possible way because so far we can use Userscript to grab the header and then send to streamlink to cache. As demonstrated here: https://github.com/streamlink/streamlink/issues/5370#issuecomment-1572768132
> Would having a browser extension be possible?
> extraction from web browser should be the easiest possible way
No, I've already commented on the webserver-bridge + browser extension idea. This is pretty bad, usability-wise and implementation-wise. It would require significant changes to Streamlink and its CLI, and how everything works. The session instance would have to spin up a web server on a plugin's request, indefinitely wait for a request being made by the browser extension until a certain time threshold is reached and then continue with the data. All while needing to have a pre-defined web server port known by the web extension, which is pretty bad if it's unavailable on the system or if multiple plugin instances need to wait for an HTTP client request. There can even be more than one session instance when implementing the Streamlink Python API into an application (that is not Streamlink CLI). Inter-process communication with the web browser is a tricky beast if you want a generic solution where the application doesn't control the web browser itself.
On top of all that, it would require the user to launch the browser manually, have the extension installed and updated, open the stream URL there and then close the tab/browser again without properly knowing when the data was found and transmitted. This all is ridiculously stupid for a single plugin. The extension would also need to work in each browser and would need to be actively maintained and properly deployed. This idea might make sense as a separate project for fetching generic stream URLs and launching Streamlink with the right parameters.
> Would it be able to support more browsers?
If you worry about support for non-Chromium-based browsers, then you'll have to wait, because getting the basic thing to work is the bigger priority. FF for example does have another communication protocol and on top of that, every browser is moving towards the W3C bidi webdriver protocol anyway which replaces CDP.
I don't want to discuss this further because this really doesn't belong into this thread. If you believe that this is an easy solution, then go ahead and submit a pull request or open another issue/discussion.
Proof of concept for minimal page to acquire tokens: https://hakkin.github.io/twitchintegrity/
https://github.com/Hakkin/twitchintegrity/blob/main/script.js
`client_ip`, `device_id` and `user_id` are signed/verified in the token, so they need to be the same in all requests using the token (`device_id` being whatever is specified in `X-Device-Id` header when fetching the token and `user_id` is tied to specified OAuth token). None of the other headers seem to be verified across requests (`Client-Session-Id`/`Client-Version`/`User-Agent`, etc).
Thanks, @Hakkin.
What I'm thinking though is, how much sense does it make re-implementing the acquirement of the integrity token when you already have to use a browser in order to make it pass the bad-bot validation anyway? This solution avoids having to access the (embedded) player directly, which isn't a bad idea, because it's obviously much faster and you're going straight for the results, without having to block access to all unwanted HTTP requests (if you decide to do that). However, there are lots of potential issues and ways Twitch can prevent scripts like this.
First, this script embeds the `p.js` script with its hardcoded URL (that URL and the device token could be passed as query string parameters) onto a different site, so we'd either
- need to host this on a local webserver and be bound to a local implementation on each Streamlink release, making it difficult to deploy patches
- or create a repo like you just did and let it get hosted by GitHub, making it a hard dependency of the plugin that could get updated and fixed much quicker
The big problem though is that a different `Referer` header will always get sent and Twitch can also easily prevent execution of remote scripts like this by adding a [`Content-Security-Policy`](https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers/Content-Security-Policy/script-src) header like they already do on their embedded web player when an incorrect `parent` URL parameter is set. Easy fix for them. Then we're back to overriding HTTP responses again via the CDP (or other means). Fetching the script sources instead of loading+executing them via a `script` tag could work though to circumvent the CSP header. But since the whole point of the integrity token is doing some fingerprinting, they could easily check `/^https:\/\/(.+\.)*twitch\.tv/.test(top.location.href)` or do some random DOM checks and we're screwed. Not trying to give them some ideas though...
You're also relying heavily on the setup of the script and how it's done by their web player, by attaching specific event listeners to the document body and passing specific objects to the main configure call. That can change easily when the motivation is to prevent tools/bots to get access tokens.
So the question is once again API stability. We've already been relying on their non-stable GQL API for a while, so this whole idea actually might not be a big problem and it'd allow us to get the token much faster.
Is RemoteWebDriver an option? Would hosting the Browser in a container (docker.io/selenium/standalone-firefox) work?
As explained, using the webdriver interface is not an option.
See the progress here: #5380 (already referenced above) | 2023-07-05T13:06:51 |
streamlink/streamlink | 5,436 | streamlink__streamlink-5436 | [
"5435"
] | e9500dff88ae9e4eb83a74b11c6ed4a6be0b460d | diff --git a/src/streamlink/plugins/mitele.py b/src/streamlink/plugins/mitele.py
--- a/src/streamlink/plugins/mitele.py
+++ b/src/streamlink/plugins/mitele.py
@@ -109,6 +109,8 @@ def _get_streams(self):
log.warning("Stream may be protected by DRM")
continue
cdn_token = tokens.get(stream["lid"], {}).get("cdn", "")
+ if not cdn_token:
+ continue
qsd = parse_qsd(cdn_token)
urls.add(update_qsd(stream["stream"], qsd, quote_via=lambda string, *_, **__: string))
| plugins.mitele: 403 Client Error: Missing hdnts for url
### Checklist
- [X] This is a plugin issue and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest stable release
### Description
The channel "Boing" on Mitele is not working with the plugin anymore...
### Debug log
```text
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.11.3
[cli][debug] Streamlink: 5.5.1
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.5.7
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.2
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.17
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.29.0
[cli][debug] urllib3: 1.26.15
[cli][debug] websocket-client: 1.5.1
[cli][debug] Arguments:
[cli][debug] url=https://www.mitele.es/directo/boing/
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --locale=es_ES
[cli][debug] --player-external-http=True
[cli][debug] --player-external-http-port=339
[cli][debug] --hls-audio-select=['*']
[cli][debug] --ffmpeg-ffmpeg=C:\Program Files\Streamlink\ffmpeg\ffmpeg.exe
[cli][debug] --ffmpeg-copyts=True
[cli][debug] --http-proxy=http://AsTJems3WD4V4HgrSTWWkJm9:[email protected]:8080/
[cli][info] Found matching plugin mitele for URL https://www.mitele.es/directo/boing/
[utils.l10n][debug] Language code: es_ES
error: Unable to open URL: https://livek.mediaset.es/orilinear31/live/linear31/playlist/playlist.isml/ctv.m3u8 (403 Client Error: Missing hdnts for url: https://livek.mediaset.es/orilinear31/live/linear31/playlist/playlist.isml/ctv.m3u8)
```
| 2023-07-12T10:47:28 |
||
streamlink/streamlink | 5,443 | streamlink__streamlink-5443 | [
"5442"
] | d89c7967402156368b81f668c6ab4ebfac42d642 | diff --git a/src/streamlink/plugins/rtvs.py b/src/streamlink/plugins/rtvs.py
--- a/src/streamlink/plugins/rtvs.py
+++ b/src/streamlink/plugins/rtvs.py
@@ -6,47 +6,52 @@
"""
import re
+from urllib.parse import urlparse
from streamlink.plugin import Plugin, pluginmatcher
from streamlink.plugin.api import validate
from streamlink.stream.hls import HLSStream
-from streamlink.utils.parse import parse_json
@pluginmatcher(re.compile(
- r"https?://www\.rtvs\.sk/televizia/live-[\w-]+",
+ r"https?://www\.rtvs\.sk/televizia/(?:live-|sport)",
))
class Rtvs(Plugin):
- _re_channel_id = re.compile(r"'stream':\s*'live-(\d+)'")
-
def _get_streams(self):
- res = self.session.http.get(self.url)
- m = self._re_channel_id.search(res.text)
- if not m:
+ channel = self.session.http.get(self.url, schema=validate.Schema(
+ validate.parse_html(),
+ validate.xml_xpath_string(".//iframe[@id='player_live']//@src"),
+ validate.url(path=validate.startswith("/embed/live/")),
+ validate.transform(lambda embed: urlparse(embed).path[len("/embed/live/"):]),
+ ))
+ if not channel:
return
- res = self.session.http.get(
+ videos = self.session.http.get(
"https://www.rtvs.sk/json/live5f.json",
params={
- "c": m.group(1),
+ "c": channel,
"b": "mozilla",
"p": "win",
"f": "0",
"d": "1",
},
+ schema=validate.Schema(
+ validate.parse_json(),
+ {
+ "clip": {
+ "sources": [{
+ "src": validate.url(),
+ "type": str,
+ }],
+ },
+ },
+ validate.get(("clip", "sources")),
+ validate.filter(lambda n: n["type"] == "application/x-mpegurl"),
+ ),
)
- videos = parse_json(res.text, schema=validate.Schema({
- "clip": {
- "sources": [{
- "src": validate.url(),
- "type": str,
- }],
- }},
- validate.get(("clip", "sources")),
- validate.filter(lambda n: n["type"] == "application/x-mpegurl"),
- ))
for video in videos:
- yield from HLSStream.parse_variant_playlist(self.session, video["src"]).items()
+ return HLSStream.parse_variant_playlist(self.session, video["src"])
__plugin__ = Rtvs
| diff --git a/tests/plugins/test_rtvs.py b/tests/plugins/test_rtvs.py
--- a/tests/plugins/test_rtvs.py
+++ b/tests/plugins/test_rtvs.py
@@ -6,13 +6,17 @@ class TestPluginCanHandleUrlRtvs(PluginCanHandleUrl):
__plugin__ = Rtvs
should_match = [
- "http://www.rtvs.sk/televizia/live-1",
- "http://www.rtvs.sk/televizia/live-2",
- "http://www.rtvs.sk/televizia/live-o",
+ "https://www.rtvs.sk/televizia/live-1",
+ "https://www.rtvs.sk/televizia/live-2",
"https://www.rtvs.sk/televizia/live-3",
+ "https://www.rtvs.sk/televizia/live-o",
"https://www.rtvs.sk/televizia/live-rtvs",
+ "https://www.rtvs.sk/televizia/live-nr-sr",
+ "https://www.rtvs.sk/televizia/sport",
]
should_not_match = [
"http://www.rtvs.sk/",
+ "http://www.rtvs.sk/televizia/archiv",
+ "http://www.rtvs.sk/televizia/program",
]
| plugins.rtvs: No playable streams found on
### Checklist
- [X] This is a plugin issue and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest build from the master branch
### Description
rtvs plugin - stream not work
### Debug log
```text
PS C:\Users\My> streamlink https://www.rtvs.sk/televizia/live-24 --loglevel debug
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.11.2
[cli][debug] Streamlink: 5.3.1
[cli][debug] Dependencies:
[cli][debug] certifi: 2022.12.7
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.2
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.17
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.28.2
[cli][debug] urllib3: 1.26.14
[cli][debug] websocket-client: 1.5.1
[cli][debug] Arguments:
[cli][debug] url=https://www.rtvs.sk/televizia/live-24
[cli][debug] --loglevel=debug
[cli][debug] --ffmpeg-ffmpeg=C:\Program Files\Streamlink\ffmpeg\ffmpeg.exe
[cli][info] Found matching plugin rtvs for URL https://www.rtvs.sk/televizia/live-24
error: No playable streams found on this URL: https://www.rtvs.sk/televizia/live-24
```
| 2023-07-15T11:21:44 |
|
streamlink/streamlink | 5,444 | streamlink__streamlink-5444 | [
"5441"
] | d89c7967402156368b81f668c6ab4ebfac42d642 | diff --git a/src/streamlink/plugins/lrt.py b/src/streamlink/plugins/lrt.py
--- a/src/streamlink/plugins/lrt.py
+++ b/src/streamlink/plugins/lrt.py
@@ -4,34 +4,42 @@
$type live
"""
-import logging
import re
from streamlink.plugin import Plugin, pluginmatcher
+from streamlink.plugin.api import validate
from streamlink.stream.hls import HLSStream
-log = logging.getLogger(__name__)
-
-
@pluginmatcher(re.compile(
r"https?://(?:www\.)?lrt\.lt/mediateka/tiesiogiai/",
))
class LRT(Plugin):
- _video_id_re = re.compile(r"""var\svideo_id\s*=\s*["'](?P<video_id>\w+)["']""")
- API_URL = "https://www.lrt.lt/servisai/stream_url/live/get_live_url.php?channel={0}"
-
def _get_streams(self):
- page = self.session.http.get(self.url)
- m = self._video_id_re.search(page.text)
- if m:
- video_id = m.group("video_id")
- data = self.session.http.get(self.API_URL.format(video_id)).json()
- hls_url = data["response"]["data"]["content"]
-
- yield from HLSStream.parse_variant_playlist(self.session, hls_url).items()
- else:
- log.debug("No match for video_id regex")
+ token_url = self.session.http.get(self.url, schema=validate.Schema(
+ re.compile(r"""var\s+tokenURL\s*=\s*(?P<q>["'])(?P<url>https://\S+)(?P=q)"""),
+ validate.none_or_all(validate.get("url")),
+ ))
+ if not token_url:
+ return
+
+ hls_url = self.session.http.get(token_url, schema=validate.Schema(
+ validate.parse_json(),
+ {
+ "response": {
+ "data": {
+ "content": validate.all(
+ str,
+ validate.transform(lambda url: url.strip()),
+ validate.url(path=validate.endswith(".m3u8")),
+ ),
+ },
+ },
+ },
+ validate.get(("response", "data", "content")),
+ ))
+
+ return HLSStream.parse_variant_playlist(self.session, hls_url)
__plugin__ = LRT
| plugins.lrt: stream is reported Forbidden (though plays if opened manually)
### Checklist
- [X] This is a plugin issue and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest stable release
### Description
When trying to open https://www.lrt.lt/mediateka/tiesiogiai/lrt-televizija or https://www.lrt.lt/mediateka/tiesiogiai/lrt-plius, an error is reported (see the log below).
However, if I try to manually pass the m3u8 URL mentioned in the error to `mpv`, like this (the URL taken from the log below, note the absence of the `%0A` at the end of it):
mpv https://af5dcb595ac445ab94d7da3af2ebb360.dlvr1.net/lrt_hd/master.m3u8?RxKc3mPWTMxjM1SuDkHZeW1Fw3jEx0oqyryrSQODiHo-Bs31UZVEBEPkLtrdbPKVKrlorJgTLUnSwqks_5Y1QrSQRYfbtlWddOuLrpnY9-kuyM_3QE_yBbqwzhre
...then, after a few ffmpeg errors and warnings, it does open.
The error started to appear a few days ago, worked perfectly before that (so, probably, they changed something at their side).
Thanks.
### Debug log
```text
[cli][debug] OS: Linux-5.15.0-76-generic-x86_64-with-glibc2.35
[cli][debug] Python: 3.11.3
[cli][debug] Streamlink: 5.5.1
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.5.7
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.2
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.18.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] urllib3: 2.0.2
[cli][debug] websocket-client: 1.5.2
[cli][debug] Arguments:
[cli][debug] url=https://www.lrt.lt/mediateka/tiesiogiai/lrt-televizija
[cli][debug] --loglevel=debug
[cli][info] Found matching plugin lrt for URL https://www.lrt.lt/mediateka/tiesiogiai/lrt-televizija
[utils.l10n][debug] Language code: en_US
error: Unable to open URL: https://af5dcb595ac445ab94d7da3af2ebb360.dlvr1.net/lrt_hd/master.m3u8?RxKc3mPWTMxjM1SuDkHZeW1Fw3jEx0oqyryrSQODiHo-Bs31UZVEBEPkLtrdbPKVKrlorJgTLUnSwqks_5Y1QrSQRYfbtlWddOuLrpnY9-kuyM_3QE_yBbqwzhre
(403 Client Error: Forbidden for url: https://af5dcb595ac445ab94d7da3af2ebb360.dlvr1.net/lrt_hd/master.m3u8?RxKc3mPWTMxjM1SuDkHZeW1Fw3jEx0oqyryrSQODiHo-Bs31UZVEBEPkLtrdbPKVKrlorJgTLUnSwqks_5Y1QrSQRYfbtlWddOuLrpnY9-kuyM_3QE_yBbqwzhre%0A)
```
| 2023-07-15T11:38:25 |
||
streamlink/streamlink | 5,477 | streamlink__streamlink-5477 | [
"5131"
] | a1e2886e17e09f582281200eaf6f0c6d35394c19 | diff --git a/src/streamlink/plugins/atresplayer.py b/src/streamlink/plugins/atresplayer.py
--- a/src/streamlink/plugins/atresplayer.py
+++ b/src/streamlink/plugins/atresplayer.py
@@ -7,7 +7,6 @@
import logging
import re
-from urllib.parse import urlparse
from streamlink.plugin import Plugin, pluginmatcher
from streamlink.plugin.api import validate
@@ -20,35 +19,33 @@
@pluginmatcher(re.compile(
- r"https?://(?:www\.)?atresplayer\.com/",
+ r"https?://(?:www\.)?atresplayer\.com/directos/.+",
))
class AtresPlayer(Plugin):
+ _channels_api_url = "https://api.atresplayer.com/client/v1/info/channels"
+ _player_api_url = "https://api.atresplayer.com/player/v1/live/{channel_id}"
+
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.url = update_scheme("https://", f"{self.url.rstrip('/')}/")
def _get_streams(self):
- path = urlparse(self.url).path
- api_url = self.session.http.get(self.url, schema=validate.Schema(
- re.compile(r"""window.__PRELOADED_STATE__\s*=\s*({.*?});""", re.DOTALL),
- validate.none_or_all(
- validate.get(1),
- validate.parse_json(),
- {"links": {path: {"href": validate.url()}}},
- validate.get(("links", path, "href")),
- ),
- ))
- if not api_url:
- return
- log.debug(f"API URL: {api_url}")
-
- player_api_url = self.session.http.get(api_url, schema=validate.Schema(
+ channel_path = f"/{self.url.split('/')[-2]}/"
+ channel_data = self.session.http.get(self._channels_api_url, schema=validate.Schema(
validate.parse_json(),
- {"urlVideo": validate.url()},
- validate.get("urlVideo"),
+ [{
+ "id": str,
+ "link": {"url": str},
+ }],
+ validate.filter(lambda item: item["link"]["url"] == channel_path),
))
+ if not channel_data:
+ return
+ channel_id = channel_data[0]["id"]
+ player_api_url = self._player_api_url.format(channel_id=channel_id)
log.debug(f"Player API URL: {player_api_url}")
+
sources = self.session.http.get(player_api_url, acceptable_status=(200, 403), schema=validate.Schema(
validate.parse_json(),
validate.any(
| diff --git a/tests/plugins/test_atresplayer.py b/tests/plugins/test_atresplayer.py
--- a/tests/plugins/test_atresplayer.py
+++ b/tests/plugins/test_atresplayer.py
@@ -10,9 +10,12 @@ class TestPluginCanHandleUrlAtresPlayer(PluginCanHandleUrl):
__plugin__ = AtresPlayer
should_match = [
- "http://www.atresplayer.com/directos/antena3/",
- "http://www.atresplayer.com/directos/lasexta/",
"https://www.atresplayer.com/directos/antena3/",
+ "https://www.atresplayer.com/directos/lasexta/",
+ "https://www.atresplayer.com/directos/antena3-internacional/",
+ ]
+
+ should_not_match = [
"https://www.atresplayer.com/flooxer/programas/unas/temporada-1/dario-eme-hache-sindy-takanashi-entrevista_123/",
]
| plugins.atresplayer: no playable streams found
### Checklist
- [X] This is a plugin issue and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest stable release
### Description
It only happens with this link (nova), not with others.
### Debug log
```text
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.11.1
[cli][debug] Streamlink: 5.2.1
[cli][debug] Dependencies:
[cli][debug] certifi: 2022.12.7
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.2
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.16.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.28.2
[cli][debug] urllib3: 1.26.14
[cli][debug] websocket-client: 1.4.2
[cli][debug] Arguments:
[cli][debug] url=https://www.atresplayer.com/directos/nova/
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --ffmpeg-ffmpeg=C:\Program Files\Streamlink\ffmpeg\ffmpeg.exe
[cli][info] Found matching plugin atresplayer for URL https://www.atresplayer.com/directos/nova/
[plugins.atresplayer][debug] API URL: https://api.atresplayer.com/client/v1/page/live/5a6a180b7ed1a834493ebf6e
[plugins.atresplayer][debug] Player API URL: https://api.atresplayer.com/player/v1/live/5a6a180b7ed1a834493ebf6e
[plugins.atresplayer][debug] Stream source: https://directo.atresmedia.com/d99c691686362f555c21feb4d596063ae356e2b4_1675105307/geonova/master.m3u8 (application/vnd.apple.mpegurl)
[utils.l10n][debug] Language code: es_ES
[cli][info] Available streams: 360p (worst), 480p, 720p, 1080p (best)
[cli][info] Opening stream: 1080p (hls)
[cli][info] Starting player: "C:\Program Files\VideoLAN\VLC\vlc.exe"
[stream.hls][debug] Reloading playlist
[cli][debug] Pre-buffering 8192 bytes
[stream.hls][debug] First Sequence: 4166611075; Last Sequence: 4166611078
[stream.hls][debug] Start offset: 0; Duration: None; Start Sequence: 4166611076; End Sequence: None
[stream.hls][debug] Adding segment 4166611076 to queue
[stream.hls][debug] Adding segment 4166611077 to queue
[stream.hls][debug] Adding segment 4166611078 to queue
[stream.hls][error] Failed to fetch segment 4166611076: Unable to open URL: https://directo.atresmedia.com/d99c691686362f555c21feb4d596063ae356e2b4_1675105307/geonova/2/br4/220629065114/92561/br4_166611076.ts (404 Client Error: Not Found for url: https://directo.atresmedia.com/d99c691686362f555c21feb4d596063ae356e2b4_1675105307/geonova/2/br4/220629065114/92561/br4_166611076.ts)
[stream.hls][error] Failed to fetch segment 4166611077: Unable to open URL: https://directo.atresmedia.com/d99c691686362f555c21feb4d596063ae356e2b4_1675105307/geonova/2/br4/220629065114/92561/br4_166611077.ts (404 Client Error: Not Found for url: https://directo.atresmedia.com/d99c691686362f555c21feb4d596063ae356e2b4_1675105307/geonova/2/br4/220629065114/92561/br4_166611077.ts)
[stream.hls][error] Failed to fetch segment 4166611078: Unable to open URL: https://directo.atresmedia.com/d99c691686362f555c21feb4d596063ae356e2b4_1675105307/geonova/2/br4/220629065114/92561/br4_166611078.ts (404 Client Error: Not Found for url: https://directo.atresmedia.com/d99c691686362f555c21feb4d596063ae356e2b4_1675105307/geonova/2/br4/220629065114/92561/br4_166611078.ts)
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Reloading playlist
[stream.segmented][debug] Closing worker thread
[stream.segmented][debug] Closing writer thread
[stream.segmented][debug] Closing writer thread
[cli][error] Try 1/1: Could not open stream <HLSStream ['hls', 'https://directo.atresmedia.com/d99c691686362f555c21feb4d596063ae356e2b4_1675105307/geonova/bitrate_4.m3u8', 'https://directo.atresmedia.com/d99c691686362f555c21feb4d596063ae356e2b4_1675105307/geonova/master.m3u8']> (Failed to read data from stream: Read timeout)
error: Could not open stream <HLSStream ['hls', 'https://directo.atresmedia.com/d99c691686362f555c21feb4d596063ae356e2b4_1675105307/geonova/bitrate_4.m3u8', 'https://directo.atresmedia.com/d99c691686362f555c21feb4d596063ae356e2b4_1675105307/geonova/master.m3u8']>, tried 1 times, exiting
[cli][info] Closing currently open stream...
```
| The issue now is on all atresplayer channels. You need to register for free (using a vpn it works). A plugin update entering a username and password should be working.
They have returned it. Everything continues as before ('nova' not works).
Any news for NOVA ?
Still Not Working on 5.3.1 version.
Still not working on 5.4.0
```text
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.11.3
[cli][debug] Streamlink: 5.4.0
[cli][debug] Dependencies:
[cli][debug] certifi: 2022.12.7
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.2
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.17
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.28.2
[cli][debug] urllib3: 1.26.15
[cli][debug] websocket-client: 1.5.1
[cli][debug] Arguments:
[cli][debug] url=https://www.atresplayer.com/directos/nova/
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --ffmpeg-ffmpeg=C:\Program Files\Streamlink\ffmpeg\ffmpeg.exe
[cli][info] Found matching plugin atresplayer for URL https://www.atresplayer.com/directos/nova/
[plugins.atresplayer][debug] API URL: https://api.atresplayer.com/client/v1/page/live/5a6a180b7ed1a834493ebf6e
[plugins.atresplayer][debug] Player API URL: https://api.atresplayer.com/player/v1/live/5a6a180b7ed1a834493ebf6e
[plugins.atresplayer][debug] Stream source: https://directo.atresmedia.com/c5b7057f9fcd5dd72d53fd60876e42cd486b965d_1681784389/geonova/master.m3u8 (application/vnd.apple.mpegurl)
[utils.l10n][debug] Language code: es_ES
[cli][info] Available streams: 360p (worst), 480p, 720p, 1080p (best)
[cli][info] Opening stream: 1080p (hls)
[cli][info] Starting player: "C:\Program Files\VideoLAN\VLC\vlc.exe"
[stream.hls][debug] Reloading playlist
[cli][debug] Pre-buffering 8192 bytes
[stream.hls][debug] First Sequence: 4166611075; Last Sequence: 4166611078
[stream.hls][debug] Start offset: 0; Duration: None; Start Sequence: 4166611076; End Sequence: None
[stream.hls][debug] Adding segment 4166611076 to queue
[stream.hls][debug] Adding segment 4166611077 to queue
[stream.hls][debug] Adding segment 4166611078 to queue
[stream.hls][error] Failed to fetch segment 4166611076: Unable to open URL: https://directo.atresmedia.com/c5b7057f9fcd5dd72d53fd60876e42cd486b965d_1681784389/geonova/2/br4/220629065114/92561/br4_166611076.ts (404 Client Error: Not Found for url: https://directo.atresmedia.com/c5b7057f9fcd5dd72d53fd60876e42cd486b965d_1681784389/geonova/2/br4/220629065114/92561/br4_166611076.ts)
[stream.hls][error] Failed to fetch segment 4166611077: Unable to open URL: https://directo.atresmedia.com/c5b7057f9fcd5dd72d53fd60876e42cd486b965d_1681784389/geonova/2/br4/220629065114/92561/br4_166611077.ts (404 Client Error: Not Found for url: https://directo.atresmedia.com/c5b7057f9fcd5dd72d53fd60876e42cd486b965d_1681784389/geonova/2/br4/220629065114/92561/br4_166611077.ts)
[stream.hls][error] Failed to fetch segment 4166611078: Unable to open URL: https://directo.atresmedia.com/c5b7057f9fcd5dd72d53fd60876e42cd486b965d_1681784389/geonova/2/br4/220629065114/92561/br4_166611078.ts (404 Client Error: Not Found for url: https://directo.atresmedia.com/c5b7057f9fcd5dd72d53fd60876e42cd486b965d_1681784389/geonova/2/br4/220629065114/92561/br4_166611078.ts)
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Reloading playlist
[stream.segmented][debug] Closing worker thread
[stream.segmented][debug] Closing writer thread
[stream.segmented][debug] Closing writer thread
[cli][error] Try 1/1: Could not open stream <HLSStream ['hls', 'https://directo.atresmedia.com/c5b7057f9fcd5dd72d53fd60876e42cd486b965d_1681784389/geonova/bitrate_4.m3u8', 'https://directo.atresmedia.com/c5b7057f9fcd5dd72d53fd60876e42cd486b965d_1681784389/geonova/master.m3u8']> (Failed to read data from stream: Read timeout)
error: Could not open stream <HLSStream ['hls', 'https://directo.atresmedia.com/c5b7057f9fcd5dd72d53fd60876e42cd486b965d_1681784389/geonova/bitrate_4.m3u8', 'https://directo.atresmedia.com/c5b7057f9fcd5dd72d53fd60876e42cd486b965d_1681784389/geonova/master.m3u8']>, tried 1 times, exiting
[cli][info] Closing currently open stream...
```
> Still not working on 5.4.0
Yes, because as you can clearly see in the changelog, nobody has submitted a pull request with a fix yet.
This site, as well as other Spanish TV streaming sites, is aggrssively blocking VPNs, which makes fixing this unnecessarily difficult. If you want this fixed, submit a PR yourself with the right changes. This is a free and open source software project, so you're not reliant on any maintainers fixing this for you.
Since the HLS playlist seems to get resolved correctly, but segment requests are failing, this suggests the following two things:
1. Either segment URLs require additional query string parameters or a URL translation of some kind (custom HLS logic)
2. or the HLS playlist URL requires certain query string parameters which are propagated to the segment URLs automatically
This plugin here has custom HLS logic which replaces segment URLs:
https://github.com/streamlink/streamlink/blob/5.4.0/src/streamlink/plugins/ltv_lsm_lv.py
you just need a spanish IP, no account needed for now : it's working fine once you're connected to a spain vpn server without any account to register.
they changed the links today :
https://www.atresplayer.com/directos/{channelnames with token: antena3, lasexta, neox, nova, mega, atreseries, canal-flooxer}
were pointing before to
https://directo.atresmedia.com/{token,etc.}
now they point to
https://atres-live.atresmedia.com/{token,etc.}
They also added some FAST channels without token if you want to add them in the atresplayer plugin :
https://www.atresplayer.com/directos/canal-kidz/
pointing to
https://fast-channels.atresmedia.com/648c271d2bfab0e4177a0d61/648c271d2bfab0e4177a0d61.m3u8
https://www.atresplayer.com/directos/aqui-no-hay-quien-viva/
pointing to
https://fast-channels.atresmedia.com/648ef3951756b0e425af83cc/648ef3951756b0e425af83cc.m3u8
https://www.atresplayer.com/directos/el-hormiguero/
pointing to
https://fast-channels.atresmedia.com/648ef5882bfab0e4627e0d61/648ef5882bfab0e4627e0d61.m3u8
https://www.atresplayer.com/directos/foq/
pointing to
https://fast-channels.atresmedia.com/648ef50a2bfab0e4607e0d61/648ef50a2bfab0e4607e0d61.m3u8
https://www.atresplayer.com/directos/equipo-de-investigacion/
pointing to
https://fast-channels.atresmedia.com/648ef5551756b0e429af83cc/648ef5551756b0e429af83cc.m3u8
https://www.atresplayer.com/directos/el-club-de-la-comedia/
pointing to
https://fast-channels.atresmedia.com/648f47f7a2ffb0e40aeff3ad/648f47f7a2ffb0e40aeff3ad.m3u8
https://www.atresplayer.com/directos/clasicos/
pointing to
https://fast-channels.atresmedia.com/648ef12c2bfab0e4507e0d61/648ef12c2bfab0e4507e0d61.m3u8
https://www.atresplayer.com/directos/multicine/
pointing to
https://fast-channels.atresmedia.com/648ef18c1756b0e41daf83cc/648ef18c1756b0e41daf83cc.m3u8
https://www.atresplayer.com/directos/comedia/
pointing to
https://fast-channels.atresmedia.com/648ef23d2bfab0e4557e0d61/648ef23d2bfab0e4557e0d61.m3u8 | 2023-08-03T12:52:26 |
streamlink/streamlink | 5,478 | streamlink__streamlink-5478 | [
"5476"
] | 4a234ff76a73ad238eea8470267366592963ad50 | diff --git a/src/streamlink/session.py b/src/streamlink/session.py
--- a/src/streamlink/session.py
+++ b/src/streamlink/session.py
@@ -241,6 +241,7 @@ def __init__(
"hls-duration": None,
"hls-playlist-reload-attempts": 3,
"hls-playlist-reload-time": "default",
+ "hls-segment-queue-threshold": 3,
"hls-segment-stream-data": False,
"hls-segment-ignore-names": [],
"hls-segment-key-uri": None,
@@ -405,6 +406,10 @@ def set_option(self, key: str, value: Any) -> None:
- ``segment``: duration of the last segment
- ``live-edge``: sum of segment durations of the ``hls-live-edge`` value minus one
- ``default``: the playlist's target duration
+ * - hls-segment-queue-threshold
+ - ``float``
+ - ``3``
+ - Factor of the playlist's targetduration which sets the threshold for stopping early on missing segments
* - hls-segment-stream-data
- ``bool``
- ``False``
diff --git a/src/streamlink/stream/hls.py b/src/streamlink/stream/hls.py
--- a/src/streamlink/stream/hls.py
+++ b/src/streamlink/stream/hls.py
@@ -151,7 +151,7 @@ def create_request_params(self, num: int, segment: Union[Segment, Map], is_map:
return request_params
- def put(self, sequence: Sequence):
+ def put(self, sequence: Optional[Sequence]):
if self.closed:
return
@@ -289,7 +289,7 @@ class HLSStreamWorker(SegmentedStreamWorker):
writer: "HLSStreamWriter"
stream: "HLSStream"
- SEGMENT_QUEUE_TIMING_THRESHOLD_FACTOR = 2
+ SEGMENT_QUEUE_TIMING_THRESHOLD_MIN = 5.0
def __init__(self, *args, **kwargs) -> None:
super().__init__(*args, **kwargs)
@@ -304,6 +304,7 @@ def __init__(self, *args, **kwargs) -> None:
self.playlist_reload_time: float = 6
self.playlist_reload_time_override = self.session.options.get("hls-playlist-reload-time")
self.playlist_reload_retries = self.session.options.get("hls-playlist-reload-attempts")
+ self.segment_queue_timing_threshold_factor = self.session.options.get("hls-segment-queue-threshold")
self.live_edge = self.session.options.get("hls-live-edge")
self.duration_offset_start = int(self.stream.start_offset + (self.session.options.get("hls-start-offset") or 0))
self.duration_limit = self.stream.duration or (
@@ -401,7 +402,13 @@ def valid_sequence(self, sequence: Sequence) -> bool:
return sequence.num >= self.playlist_sequence
def _segment_queue_timing_threshold_reached(self) -> bool:
- threshold = self.playlist_targetduration * self.SEGMENT_QUEUE_TIMING_THRESHOLD_FACTOR
+ if self.segment_queue_timing_threshold_factor <= 0:
+ return False
+
+ threshold = max(
+ self.SEGMENT_QUEUE_TIMING_THRESHOLD_MIN,
+ self.playlist_targetduration * self.segment_queue_timing_threshold_factor,
+ )
if now() <= self.playlist_sequences_last + timedelta(seconds=threshold):
return False
diff --git a/src/streamlink_cli/argparser.py b/src/streamlink_cli/argparser.py
--- a/src/streamlink_cli/argparser.py
+++ b/src/streamlink_cli/argparser.py
@@ -953,6 +953,24 @@ def build_parser():
Default is default.
""",
)
+ transport_hls.add_argument(
+ "--hls-segment-queue-threshold",
+ metavar="FACTOR",
+ type=num(float, ge=0),
+ help="""
+ The multiplication factor of the HLS playlist's target duration after which the stream will be stopped early
+ if no new segments were queued after refreshing the playlist (multiple times). The target duration defines the
+ maximum duration a single segment can have, meaning new segments must be available during this time frame,
+ otherwise playback issues can occur.
+
+ The intention of this queue threshold is to be able to stop early when the end of a stream doesn't get
+ announced by the server, so Streamlink doesn't have to wait until a read-timeout occurs. See --stream-timeout.
+
+ Set to ``0`` to disable.
+
+ Default is 3.
+ """,
+ )
transport_hls.add_argument(
"--hls-segment-ignore-names",
metavar="NAMES",
@@ -1376,6 +1394,7 @@ def build_parser():
("hls_duration", "hls-duration", None),
("hls_playlist_reload_attempts", "hls-playlist-reload-attempts", None),
("hls_playlist_reload_time", "hls-playlist-reload-time", None),
+ ("hls_segment_queue_threshold", "hls-segment-queue-threshold", None),
("hls_segment_stream_data", "hls-segment-stream-data", None),
("hls_segment_ignore_names", "hls-segment-ignore-names", None),
("hls_segment_key_uri", "hls-segment-key-uri", None),
| diff --git a/tests/stream/test_hls.py b/tests/stream/test_hls.py
--- a/tests/stream/test_hls.py
+++ b/tests/stream/test_hls.py
@@ -218,7 +218,7 @@ def test_segment_queue_timing_threshold_reached(self) -> None:
frozen_time.tick(ONE_SECOND)
self.await_playlist_reload(1)
- assert worker.handshake_wait.wait_ready(1), "Arrives at first wait() call"
+ assert worker.handshake_wait.wait_ready(1), "Arrives at wait() call #1"
assert worker.playlist_sequence == 1, "Updates the sequence number"
assert worker.playlist_sequences_last == EPOCH + ONE_SECOND, "Updates the last queue time"
assert worker.playlist_targetduration == 5.0
@@ -228,30 +228,117 @@ def test_segment_queue_timing_threshold_reached(self) -> None:
self.await_playlist_wait(1)
self.await_playlist_reload(1)
- assert worker.handshake_wait.wait_ready(1), "Arrives at second wait() call"
+ assert worker.handshake_wait.wait_ready(1), "Arrives at wait() call #2"
assert worker.playlist_sequence == 2, "Updates the sequence number again"
assert worker.playlist_sequences_last == EPOCH + ONE_SECOND + targetduration, "Updates the last queue time again"
assert worker.playlist_targetduration == 5.0
+ for num in range(3, 6):
+ # trigger next reload when the target duration has passed
+ frozen_time.tick(targetduration)
+ self.await_playlist_wait(1)
+ self.await_playlist_reload(1)
+
+ assert worker.handshake_wait.wait_ready(1), f"Arrives at wait() call #{num}"
+ assert worker.playlist_sequence == 2, "Sequence number is unchanged"
+ assert worker.playlist_sequences_last == EPOCH + ONE_SECOND + targetduration, "Last queue time is unchanged"
+ assert worker.playlist_targetduration == 5.0
+
+ assert mock_log.warning.call_args_list == []
+
# trigger next reload when the target duration has passed
frozen_time.tick(targetduration)
self.await_playlist_wait(1)
self.await_playlist_reload(1)
- assert worker.handshake_wait.wait_ready(1), "Arrives at third wait() call"
- assert worker.playlist_sequence == 2, "Sequence number is unchanged"
- assert worker.playlist_sequences_last == EPOCH + ONE_SECOND + targetduration, "Last queue time is unchanged"
- assert worker.playlist_targetduration == 5.0
+ self.await_read(read_all=True)
+ self.await_close(1)
- # trigger next reload when the target duration has passed
- frozen_time.tick(targetduration)
- self.await_playlist_wait(1)
+ assert mock_log.warning.call_args_list == [call("No new segments in playlist for more than 15.00s. Stopping...")]
+
+ def test_segment_queue_timing_threshold_reached_ignored(self) -> None:
+ thread, segments = self.subject(
+ start=False,
+ options={"hls-segment-queue-threshold": 0},
+ playlists=[
+ # no EXT-X-ENDLIST, last mocked playlist response will be repreated forever
+ Playlist(0, targetduration=5, segments=[Segment(0)]),
+ ],
+ )
+ worker: EventedHLSStreamWorker = thread.reader.worker
+ targetduration = ONE_SECOND * 5
+
+ with freezegun.freeze_time(EPOCH) as frozen_time:
+ self.start()
+
+ assert worker.handshake_reload.wait_ready(1), "Loads playlist for the first time"
+ assert worker.playlist_sequence == -1, "Initial sequence number"
+ assert worker.playlist_sequences_last == EPOCH, "Sets the initial last queue time"
+
+ # first playlist reload has taken one second
+ frozen_time.tick(ONE_SECOND)
self.await_playlist_reload(1)
- assert worker.handshake_wait.wait_ready(1), "Arrives at fourth wait() call"
- assert worker.playlist_sequence == 2, "Sequence number is unchanged"
- assert worker.playlist_sequences_last == EPOCH + ONE_SECOND + targetduration, "Last queue time is unchanged"
+ assert worker.handshake_wait.wait_ready(1), "Arrives at first wait() call"
+ assert worker.playlist_sequence == 1, "Updates the sequence number"
+ assert worker.playlist_sequences_last == EPOCH + ONE_SECOND, "Updates the last queue time"
assert worker.playlist_targetduration == 5.0
+ assert self.await_read() == self.content(segments)
+
+ # keep reloading a couple of times
+ for num in range(10):
+ frozen_time.tick(targetduration)
+ self.await_playlist_wait(1)
+ self.await_playlist_reload(1)
+
+ assert worker.handshake_wait.wait_ready(1), f"Arrives at wait() #{num + 1}"
+ assert worker.playlist_sequence == 1, "Sequence number is unchanged"
+ assert worker.playlist_sequences_last == EPOCH + ONE_SECOND, "Last queue time is unchanged"
+
+ assert self.thread.data == [], "No new data"
+ assert worker.is_alive()
+
+ # make stream end gracefully to avoid any unnecessary thread blocking
+ self.thread.reader.writer.put(None)
+
+ def test_segment_queue_timing_threshold_reached_min(self) -> None:
+ thread, segments = self.subject(
+ start=False,
+ playlists=[
+ # no EXT-X-ENDLIST, last mocked playlist response will be repreated forever
+ Playlist(0, targetduration=1, segments=[Segment(0)]),
+ ],
+ )
+ worker: EventedHLSStreamWorker = thread.reader.worker
+ targetduration = ONE_SECOND
+
+ with freezegun.freeze_time(EPOCH) as frozen_time, \
+ patch("streamlink.stream.hls.log") as mock_log:
+ self.start()
+
+ assert worker.handshake_reload.wait_ready(1), "Loads playlist for the first time"
+ assert worker.playlist_sequence == -1, "Initial sequence number"
+ assert worker.playlist_sequences_last == EPOCH, "Sets the initial last queue time"
+
+ # first playlist reload has taken one second
+ frozen_time.tick(ONE_SECOND)
+ self.await_playlist_reload(1)
+
+ assert worker.handshake_wait.wait_ready(1), "Arrives at wait() call #1"
+ assert worker.playlist_sequence == 1, "Updates the sequence number"
+ assert worker.playlist_sequences_last == EPOCH + ONE_SECOND, "Updates the last queue time"
+ assert worker.playlist_targetduration == 1.0
+
+ for num in range(2, 7):
+ # trigger next reload when the target duration has passed
+ frozen_time.tick(targetduration)
+ self.await_playlist_wait(1)
+ self.await_playlist_reload(1)
+
+ assert worker.handshake_wait.wait_ready(1), f"Arrives at wait() call #{num}"
+ assert worker.playlist_sequence == 1, "Sequence number is unchanged"
+ assert worker.playlist_sequences_last == EPOCH + ONE_SECOND, "Last queue time is unchanged"
+ assert worker.playlist_targetduration == 1.0
assert mock_log.warning.call_args_list == []
@@ -260,10 +347,7 @@ def test_segment_queue_timing_threshold_reached(self) -> None:
self.await_playlist_wait(1)
self.await_playlist_reload(1)
- self.await_read(read_all=True)
- self.await_close(1)
-
- assert mock_log.warning.call_args_list == [call("No new segments in playlist for more than 10.00s. Stopping...")]
+ assert mock_log.warning.call_args_list == [call("No new segments in playlist for more than 5.00s. Stopping...")]
def test_playlist_reload_offset(self) -> None:
thread, segments = self.subject(
| stream.hls: Could the "No new segments in playlist" judgment be longer or customizable?
### Checklist
- [X] This is a plugin issue and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest stable release
### Description
The SHOWROOM plugin in Streamlink seems to have been updated from 6.0.0 and a new feature has been introduced: to check if the segments in the playlist are exhausted or not to determine to stop streaming. Like this:
```
[download] Written 272.81 MiB to [Filename].ts (29m39s @ 145.41 KiB/s)
[stream.hls][debug] Writing segment 896 to output
[stream.hls][debug] Segment 896 complete
[download] Written 273.10 MiB to [Filename].ts (29m41s @ 143.74 KiB/s)
[stream.hls][debug] Reloading playlist
[download] Written 273.10 MiB to [Filename].ts (29m42s @ 143.74 KiB/s)
[stream.hls][debug] Reloading playlist
[download] Written 273.10 MiB to [Filename].ts (29m43s @ 143.74 KiB/s)
[stream.hls][debug] Reloading playlist
[download] Written 273.10 MiB to [Filename].ts (29m44s @ 129.23 KiB/s)
[stream.hls][debug] Reloading playlist
[download] Written 273.10 MiB to [Filename].ts (29m45s @ 114.84 KiB/s)
[stream.hls][debug] Reloading playlist
[download] Written 273.10 MiB to [Filename].ts (29m46s @ 114.84 KiB/s)
[stream.hls][debug] Reloading playlist
[download] Written 273.10 MiB to [Filename].ts (29m47s @ 114.84 KiB/s)
[stream.hls][debug] Reloading playlist
[download] Written 273.10 MiB to [Filename].ts (29m47s @ 99.49 KiB/s)
[stream.hls][warning] No new segments in playlist for more than 8.00s. Stopping...
[stream.segmented][debug] Closing worker thread
[stream.segmented][debug] Closing writer thread
[cli][info] Stream ended
[cli][info] Closing currently open stream...
```
As far as I have observed, the time above (8.00s) is not a fixed number, it could be 6/8/10s in other runs. And I have two questions:
1. I didn't see any instruction to let users to configure the time value, so could it be able to customize? I'd be very grateful if you could make this possible in further updates.
2. If you don't have the plan to make it open to customization, could you please make it a bit longer? One minute might be better for SHOWROOM, because it is not rare that SHOWROOM broadcasters happen to have network problems, which will make a short period of stop in updating playlist. And the current time can easily cause Streamlink to stop recording before the stream restarts.
### Debug log
```text
...
[download] Written 272.81 MiB to [Filename].ts (29m39s @ 145.41 KiB/s)
[stream.hls][debug] Writing segment 896 to output
[stream.hls][debug] Segment 896 complete
[download] Written 273.10 MiB to [Filename].ts (29m41s @ 143.74 KiB/s)
[stream.hls][debug] Reloading playlist
[download] Written 273.10 MiB to [Filename].ts (29m42s @ 143.74 KiB/s)
[stream.hls][debug] Reloading playlist
[download] Written 273.10 MiB to [Filename].ts (29m43s @ 143.74 KiB/s)
[stream.hls][debug] Reloading playlist
[download] Written 273.10 MiB to [Filename].ts (29m44s @ 129.23 KiB/s)
[stream.hls][debug] Reloading playlist
[download] Written 273.10 MiB to [Filename].ts (29m45s @ 114.84 KiB/s)
[stream.hls][debug] Reloading playlist
[download] Written 273.10 MiB to [Filename].ts (29m46s @ 114.84 KiB/s)
[stream.hls][debug] Reloading playlist
[download] Written 273.10 MiB to [Filename].ts (29m47s @ 114.84 KiB/s)
[stream.hls][debug] Reloading playlist
[download] Written 273.10 MiB to [Filename].ts (29m47s @ 99.49 KiB/s)
[stream.hls][warning] No new segments in playlist for more than 8.00s. Stopping...
[stream.segmented][debug] Closing worker thread
[stream.segmented][debug] Closing writer thread
[cli][info] Stream ended
[cli][info] Closing currently open stream...
```
| As you can see in the [6.0.0 changelog](https://github.com/streamlink/streamlink/releases/tag/6.0.0), this behavior was added in #5330, and it stops the streams if no new segments were queued for a while. The intention here is to stop early when the `EXT-X-ENDLIST` tag is missing, meaning the server didn't announce the end of the stream properly. In this case, Streamlink would need to wait until the stream's read-timeout gets triggered.
The max time of missing segments depends on the playlist's targetduration. Streamlink waits for at most twice this duration for new segments to be queued while polling the playlist.
We can make it customizable though.
> The intention here is to stop early when the `EXT-X-ENDLIST` tag is missing, meaning the server didn't announce the end of the stream properly. In this case, Streamlink would need to wait until the stream's read-timeout gets triggered.
Thank you for your detailed instructions. Streams on SHOWROOM never declared this tag when no new segments was appended. So I understand it's necessary to make this change.
> We can make it customizable though.
Really appreciate and look forward to it. Thank you so much.
+1 for this change request to make the timeout customizable/able to be overriden. This issue bit me 4x already when watching live youtube streams that had some latency.
This has nothing to do with latency. If you're having issues with your own connection to the server, then `--stream-timeout` is what you're looking for. The segment-queuing in regards to the playlist's targetduration is only relevant when the server doesn't queue new segments and thus violates the HLS protocol. This can happen when the stream ingestion from the streamer/broadcaster is unstable and the server doesn't push any filler segments
> This can happen when the stream ingestion from the streamer/broadcaster is unstable
that happens quite often in the streams that i usually watch, i had streamlink quit without the stream actually ending a few times already. an option to disable it or change the time it waits would be appreciated.
@bastimeyer I have stream_timeout set to 1200, and have never had a youtube live stream timeout before the upgrade to 6.0
And I'm definitely not having connectivity issues with youtube. My computer has an ethernet connection directly into my FIOS (1G symmetrical) router, and any temporary connection issues would not be present over several days. Let me see if I can find debug output or not. If not I will share the next time it happens.
(Sadly my debug output is gone as my computer has rebooted for required security updates)
This has nothing to do with `--stream-timeout` or any other local connectivity issues. I have already explained that.
Read #5330.
As I've said, I will add an option later to configure the new behavior.
Thanks for the pointer to the change. Yes, that change has definitely hit me in a negative way, stopping the stream before the end 4x for me so far on youtube live streams (but has not effected other non-youtube live streams) | 2023-08-03T22:09:40 |
streamlink/streamlink | 5,480 | streamlink__streamlink-5480 | [
"5461",
"5461"
] | 3a38ca2e6f66a6b11ea4d96b8d0f0f9c780f9948 | diff --git a/src/streamlink/utils/processoutput.py b/src/streamlink/utils/processoutput.py
--- a/src/streamlink/utils/processoutput.py
+++ b/src/streamlink/utils/processoutput.py
@@ -48,9 +48,9 @@ async def onoutput(callback: Callable[[int, str], Optional[bool]], streamreader:
tasks = (
loop.create_task(ontimeout()),
- loop.create_task(onexit()),
loop.create_task(onoutput(self.onstdout, process.stdout)),
loop.create_task(onoutput(self.onstderr, process.stderr)),
+ loop.create_task(onexit()),
)
try:
| diff --git a/tests/utils/test_processoutput.py b/tests/utils/test_processoutput.py
--- a/tests/utils/test_processoutput.py
+++ b/tests/utils/test_processoutput.py
@@ -217,3 +217,23 @@ async def test_onoutput_exception(event_loop: asyncio.BaseEventLoop, processoutp
assert processoutput.onstdout.call_args_list == [call(0, "foo")]
assert processoutput.onstderr.call_args_list == []
assert mock_process.kill.called
+
+
[email protected]()
+async def test_exit_before_onoutput(event_loop: asyncio.BaseEventLoop, processoutput: FakeProcessOutput, mock_process: Mock):
+ # resolve process.wait() in the onexit task immediately
+ mock_process.wait = AsyncMock(return_value=0)
+
+ # add some data to stdout, but don't actually interpret it in onstdout
+ mock_process.stdout.append(b"foo")
+ processoutput.onstdout.return_value = True
+
+ # the result of the `done` future should have already been set by the onoutput task
+ processoutput.onexit.return_value = False
+
+ result = await processoutput._run()
+
+ assert result is True
+ assert processoutput.onexit.called
+ assert processoutput.onstdout.called
+ assert mock_process.kill.called
| Unexpected FFmpeg version output while running ['/usr/bin/ffmpeg', '-version']
### Checklist
- [X] This is a bug report and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed bug reports](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22bug%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest stable release
### Description
FFmpeg binary not validated.
Streamlink version 6.0.0.0 Linux Appimage x86_64
Got this error message:
[stream.ffmpegmux][error] Could not validate FFmpeg!
[stream.ffmpegmux][error] Unexpected FFmpeg version output while running ['/usr/bin/ffmpeg', '-version']
[stream.ffmpegmux][warning] No valid FFmpeg binary was found. See the --ffmpeg-ffmpeg option.
[stream.ffmpegmux][warning] Muxing streams is unsupported! Only a subset of the available streams can be returned!
ffmpeg output:
ffmpeg -version
ffmpeg version 6.0-static https://johnvansickle.com/ffmpeg/ Copyright (c) 2000-2023 the FFmpeg developers
built with gcc 8 (Debian 8.3.0-6)
ffmpeg is in /usr/bin
### Debug log
```text
[utils.l10n][debug] Language code: en_US
[stream.ffmpegmux][error] Could not validate FFmpeg!
[stream.ffmpegmux][error] Unexpected FFmpeg version output while running ['/usr/bin/ffmpeg', '-version']
[stream.ffmpegmux][warning] No valid FFmpeg binary was found. See the --ffmpeg-ffmpeg option.
[stream.ffmpegmux][warning] Muxing streams is unsupported! Only a subset of the available streams can be returned!
[cli][info] Available streams: 234p_alt (worst), 234p, 360p_alt2, 360p_alt, 360p, 540p, 720p_alt, 720p, 1080p (best)
[cli][info] Opening stream: 720p (hls)
[cli][info] Starting player: mpv
[stream.hls][debug] Reloading playlist
[cli][debug] Pre-buffering 8192 bytes
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] First Sequence: 22258880; Last Sequence: 22259722
[stream.hls][debug] Start offset: 0; Duration: None; Start Sequence: 22259720; End Sequence: None
[stream.hls][debug] Adding segment 22259720 to queue
[stream.hls][debug] Adding segment 22259721 to queue
[stream.hls][debug] Adding segment 22259722 to queue
[stream.hls][debug] Writing segment 22259720 to output
[stream.hls][debug] Segment 22259720 complete
```
Unexpected FFmpeg version output while running ['/usr/bin/ffmpeg', '-version']
### Checklist
- [X] This is a bug report and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed bug reports](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22bug%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest stable release
### Description
FFmpeg binary not validated.
Streamlink version 6.0.0.0 Linux Appimage x86_64
Got this error message:
[stream.ffmpegmux][error] Could not validate FFmpeg!
[stream.ffmpegmux][error] Unexpected FFmpeg version output while running ['/usr/bin/ffmpeg', '-version']
[stream.ffmpegmux][warning] No valid FFmpeg binary was found. See the --ffmpeg-ffmpeg option.
[stream.ffmpegmux][warning] Muxing streams is unsupported! Only a subset of the available streams can be returned!
ffmpeg output:
ffmpeg -version
ffmpeg version 6.0-static https://johnvansickle.com/ffmpeg/ Copyright (c) 2000-2023 the FFmpeg developers
built with gcc 8 (Debian 8.3.0-6)
ffmpeg is in /usr/bin
### Debug log
```text
[utils.l10n][debug] Language code: en_US
[stream.ffmpegmux][error] Could not validate FFmpeg!
[stream.ffmpegmux][error] Unexpected FFmpeg version output while running ['/usr/bin/ffmpeg', '-version']
[stream.ffmpegmux][warning] No valid FFmpeg binary was found. See the --ffmpeg-ffmpeg option.
[stream.ffmpegmux][warning] Muxing streams is unsupported! Only a subset of the available streams can be returned!
[cli][info] Available streams: 234p_alt (worst), 234p, 360p_alt2, 360p_alt, 360p, 540p, 720p_alt, 720p, 1080p (best)
[cli][info] Opening stream: 720p (hls)
[cli][info] Starting player: mpv
[stream.hls][debug] Reloading playlist
[cli][debug] Pre-buffering 8192 bytes
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] First Sequence: 22258880; Last Sequence: 22259722
[stream.hls][debug] Start offset: 0; Duration: None; Start Sequence: 22259720; End Sequence: None
[stream.hls][debug] Adding segment 22259720 to queue
[stream.hls][debug] Adding segment 22259721 to queue
[stream.hls][debug] Adding segment 22259722 to queue
[stream.hls][debug] Writing segment 22259720 to output
[stream.hls][debug] Segment 22259720 complete
```
| You've removed parts of the debug log, so it's unclear which ffmpeg binary you've actually set.
Streamlink checks the first line of the stdout stream when running `ffmpeg -version`, as you can see here:
https://github.com/streamlink/streamlink/blob/6.0.0/src/streamlink/stream/ffmpegmux.py#L261-L267
The output of these custom-built ffmpeg binaries works just fine:
```
$ ./ffmpeg-6.0-amd64-static/ffmpeg -version 2>/dev/null | head -n1
ffmpeg version 6.0-static https://johnvansickle.com/ffmpeg/ Copyright (c) 2000-2023 the FFmpeg developers
```
Output from a temp docker container running the ffmpeg binary:
```
$ /opt/python/cp311-cp311/bin/python -m streamlink --loglevel=debug --output=/dev/null --ffmpeg-ffmpeg=/ffmpeg/ffmpeg-6.0-amd64-static/ffmpeg https://steamcommunity.com/broadcast/watch/76561199514732246 best
[cli][info] streamlink is running as root! Be careful!
[cli][debug] OS: Linux-6.4.6-1-git-x86_64-with-glibc2.17
[cli][debug] Python: 3.11.4
[cli][debug] Streamlink: 6.0.0
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.7.22
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.3
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.18.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.22.2
[cli][debug] trio-websocket: 0.10.3
[cli][debug] typing-extensions: 4.7.1
[cli][debug] urllib3: 2.0.4
[cli][debug] websocket-client: 1.6.1
[cli][debug] Arguments:
[cli][debug] url=https://steamcommunity.com/broadcast/watch/76561199514732246
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --output=/dev/null
[cli][debug] --ffmpeg-ffmpeg=/ffmpeg/ffmpeg-6.0-amd64-static/ffmpeg
[cli][info] Found matching plugin steam for URL https://steamcommunity.com/broadcast/watch/76561199514732246
[plugins.steam][debug] Getting broadcast stream: sessionid=d3e4ef22f1a58e991fc13ab4
[utils.l10n][debug] Language code: en_US
[stream.dash][debug] Available languages for DASH audio streams: und (using: und)
[cli][info] Available streams: 360p (worst), 480p, 720p, 1080p (best)
[cli][info] Opening stream: 1080p (dash)
[cli][info] Writing output to
/dev/null
[cli][debug] Checking file output
[stream.dash][debug] Opening DASH reader for: ('1', 'video', '1') - video/mp4
[stream.dash][debug] Opening DASH reader for: ('1', 'audio', '1') - audio/mp4
[stream.dash][debug] video/mp4 segment initialization: downloading (2023-07-25T22:09:33.000000Z / 2023-07-27T17:25:15.525464Z)
[stream.dash_manifest][debug] Generating segment numbers for dynamic playlist: ('1', 'video', '1')
[stream.dash_manifest][debug] Stream start: 2023-07-25 22:09:33+00:00
[stream.dash_manifest][debug] Current time: 2023-07-27 17:25:15.523858+00:00
[stream.dash_manifest][debug] Availability: 2023-07-27 17:25:09+00:00
[stream.dash_manifest][debug] presentationTimeOffset: 0:00:00; suggestedPresentationDelay: 0:00:03; minBufferTime: 0:00:03
[stream.dash_manifest][debug] segmentDuration: 2.0; segmentStart: 1; segmentOffset: 77868 (155736.523858s)
[stream.dash][debug] audio/mp4 segment initialization: downloading (2023-07-25T22:09:33.000000Z / 2023-07-27T17:25:15.528351Z)
[stream.dash_manifest][debug] Generating segment numbers for dynamic playlist: ('1', 'audio', '1')
[stream.dash_manifest][debug] Stream start: 2023-07-25 22:09:33+00:00
[stream.dash_manifest][debug] Current time: 2023-07-27 17:25:15.523858+00:00
[stream.dash_manifest][debug] Availability: 2023-07-27 17:25:09+00:00
[stream.dash_manifest][debug] presentationTimeOffset: 0:00:00; suggestedPresentationDelay: 0:00:03; minBufferTime: 0:00:03
[stream.dash_manifest][debug] segmentDuration: 2.0; segmentStart: 1; segmentOffset: 77868 (155736.523858s)
[stream.ffmpegmux][debug] ffmpeg version 6.0-static https://johnvansickle.com/ffmpeg/ Copyright (c) 2000-2023 the FFmpeg developers
[stream.ffmpegmux][debug] built with gcc 8 (Debian 8.3.0-6)
[stream.ffmpegmux][debug] configuration: --enable-gpl --enable-version3 --enable-static --disable-debug --disable-ffplay --disable-indev=sndio --disable-outdev=sndio --cc=gcc --enable-fontconfig --enable-frei0r --enable-gnutls --enable-gmp --enable-libgme --enable-gray --enable-libaom --enable-libfribidi --enable-libass --enable-libvmaf --enable-libfreetype --enable-libmp3lame --enable-libopencore-amrnb --enable-libopencore-amrwb --enable-libopenjpeg --enable-librubberband --enable-libsoxr --enable-libspeex --enable-libsrt --enable-libvorbis --enable-libopus --enable-libtheora --enable-libvidstab --enable-libvo-amrwbenc --enable-libvpx --enable-libwebp --enable-libx264 --enable-libx265 --enable-libxml2 --enable-libdav1d --enable-libxvid --enable-libzvbi --enable-libzimg
[stream.ffmpegmux][debug] libavutil 58. 2.100 / 58. 2.100
[stream.ffmpegmux][debug] libavcodec 60. 3.100 / 60. 3.100
[stream.ffmpegmux][debug] libavformat 60. 3.100 / 60. 3.100
[stream.ffmpegmux][debug] libavdevice 60. 1.100 / 60. 1.100
[stream.ffmpegmux][debug] libavfilter 9. 3.100 / 9. 3.100
[stream.ffmpegmux][debug] libswscale 7. 1.100 / 7. 1.100
[stream.ffmpegmux][debug] libswresample 4. 10.100 / 4. 10.100
[stream.ffmpegmux][debug] libpostproc 57. 1.100 / 57. 1.100
[utils.named_pipe][info] Creating pipe streamlinkpipe-53-1-9459
[utils.named_pipe][info] Creating pipe streamlinkpipe-53-2-4204
[stream.ffmpegmux][debug] ffmpeg command: /ffmpeg/ffmpeg-6.0-amd64-static/ffmpeg -nostats -y -i /tmp/streamlinkpipe-53-1-9459 -i /tmp/streamlinkpipe-53-2-4204 -c:v copy -c:a copy -copyts -f matroska pipe:1
[stream.ffmpegmux][debug] Starting copy to pipe: /tmp/streamlinkpipe-53-1-9459
[stream.ffmpegmux][debug] Starting copy to pipe: /tmp/streamlinkpipe-53-2-4204
[cli][debug] Pre-buffering 8192 bytes
[stream.dash][debug] audio/mp4 segment 77869: downloading (2023-07-27T17:25:09.000000Z / 2023-07-27T17:25:15.653844Z)
[stream.dash][debug] audio/mp4 segment initialization: completed
[stream.dash][debug] audio/mp4 segment 77870: downloading (2023-07-27T17:25:11.000000Z / 2023-07-27T17:25:15.695339Z)
[stream.dash][debug] audio/mp4 segment 77869: completed
[stream.dash][debug] audio/mp4 segment 77871: downloading (2023-07-27T17:25:13.000000Z / 2023-07-27T17:25:15.755956Z)
[stream.dash][debug] audio/mp4 segment 77870: completed
[stream.dash][debug] video/mp4 segment 77869: downloading (2023-07-27T17:25:09.000000Z / 2023-07-27T17:25:15.783191Z)
[stream.dash][debug] video/mp4 segment initialization: completed
[stream.dash][debug] audio/mp4 segment 77872: downloading (2023-07-27T17:25:15.000000Z / 2023-07-27T17:25:15.808912Z)
[stream.dash][debug] audio/mp4 segment 77871: completed
[stream.dash][debug] audio/mp4 segment 77873: waiting 1.1s (2023-07-27T17:25:17.000000Z / 2023-07-27T17:25:15.896141Z)
[stream.dash][debug] audio/mp4 segment 77872: completed
[stream.dash][debug] video/mp4 segment 77870: downloading (2023-07-27T17:25:11.000000Z / 2023-07-27T17:25:16.888916Z)
[stream.dash][debug] video/mp4 segment 77869: completed
[stream.dash][debug] audio/mp4 segment 77873: downloading (2023-07-27T17:25:17.000000Z / 2023-07-27T17:25:17.000241Z)
[cli][debug] Writing stream to output
[stream.dash][debug] audio/mp4 segment 77874: waiting 1.8s (2023-07-27T17:25:19.000000Z / 2023-07-27T17:25:17.200659Z)
[stream.dash][debug] audio/mp4 segment 77873: completed
[download] Written 592.00 KiB to /dev/null (0s) [stream.dash][debug] video/mp4 segment 77871: downloading (2023-07-27T17:25:13.000000Z / 2023-07-27T17:25:17.302594Z)
[stream.dash][debug] video/mp4 segment 77870: completed
^C
[stream.ffmpegmux][debug] Closing ffmpeg thread
[stream.segmented][debug] Closing worker thread
[stream.segmented][debug] Closing writer thread
[stream.ffmpegmux][debug] Pipe copy complete: /tmp/streamlinkpipe-53-1-9459
[stream.segmented][debug] Closing worker thread
[stream.segmented][debug] Closing writer thread
[stream.ffmpegmux][debug] Pipe copy complete: /tmp/streamlinkpipe-53-2-4204
[stream.dash][debug] audio/mp4 segment 77874: cancelled
[stream.dash][warning] video/mp4 segment 77871: aborted
[stream.ffmpegmux][debug] Closed all the substreams
[cli][info] Stream ended
Interrupted! Exiting...
[cli][info] Closing currently open stream...
```
[cli][debug] OS: Linux-3.10.0-1062.4.1.el7.x86_64-x86_64-with-glibc2.17
[cli][debug] Python: 3.11.4
[cli][debug] Streamlink: 6.0.0
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.5.7
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.2
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.18.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.22.2
[cli][debug] trio-websocket: 0.10.3
[cli][debug] typing-extensions: 4.7.1
[cli][debug] urllib3: 2.0.3
[cli][debug] websocket-client: 1.6.1
[cli][debug] Arguments:
[cli][debug] url=XXX
[cli][debug] --loglevel=debug
[cli][debug] --player=mpv
[cli][debug] --player-no-close=True
[cli][debug] --output=/dev/null
[cli][debug] --default-stream=['720p', 'best', 'vod']
[cli][debug] --ringbuffer-size=33554432
[cli][debug] --stream-segment-attempts=4
[cli][debug] --stream-segment-timeout=30.0
[cli][debug] --stream-timeout=60.0
[cli][debug] --hls-playlist-reload-attempts=4
[cli][debug] --hls-playlist-reload-time=segment
[cli][debug] --ffmpeg-ffmpeg=/usr/bin/ffmpeg
[cli][debug] --http-header=[('User-Agent', '"Mozilla/5.0 (Windows NT 6.1; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/75.0.3770.100 Safari/537.36 OPR/62.0.3331.66"'), ('User-Agent', 'Mozilla/5.0 (Windows NT 10.0; rv:114.0) Gecko/20100101 Firefox/114.0')]
[cli][debug] --twitch-disable-ads=True
[cli][debug] --twitch-disable-hosting=True
[cli][info] Found matching plugin hls for URL XXX
[plugins.hls][debug] URL=private
[utils.l10n][debug] Language code: en_US
[stream.ffmpegmux][error] Could not validate FFmpeg!
[stream.ffmpegmux][error] Unexpected FFmpeg version output while running ['/usr/bin/ffmpeg', '-version']
[stream.ffmpegmux][warning] No valid FFmpeg binary was found. See the --ffmpeg-ffmpeg option.
[stream.ffmpegmux][warning] Muxing streams is unsupported! Only a subset of the available streams can be returned!
[cli][info] Available streams: 234p_alt (worst), 234p, 360p_alt2, 360p_alt, 360p, 540p, 720p_alt, 720p, 1080p (best)
[cli][info] Opening stream: 720p (hls)
[cli][info] Writing output to
/dev/null
[cli][debug] Checking file output
[stream.hls][debug] Reloading playlist
[cli][debug] Pre-buffering 8192 bytes
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] First Sequence: 4946541; Last Sequence: 4947172
[stream.hls][debug] Start offset: 0; Duration: None; Start Sequence: 4947170; End Sequence: None
[stream.hls][debug] Adding segment 4947170 to queue
[stream.hls][debug] Adding segment 4947171 to queue
[stream.hls][debug] Adding segment 4947172 to queue
[stream.hls][debug] Writing segment 4947170 to output
[stream.hls][debug] Segment 4947170 complete
[stream.hls][debug] Writing segment 4947171 to output
[stream.hls][debug] Segment 4947171 complete
[stream.hls][debug] Writing segment 4947172 to output
[stream.hls][debug] Segment 4947172 complete
[cli][debug] Writing stream to output
[download] Written 1.36 MiB to /dev/null (4s @ 303.87 KiB/s)
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 4947173 to queue
[stream.hls][debug] Writing segment 4947173 to output
[stream.hls][debug] Segment 4947173 complete
[download] Written 1.83 MiB to /dev/null (6s @ 292.90 KiB/s)
^C
[stream.segmented][debug] Closing worker thread
[stream.segmented][debug] Closing writer thread
[cli][info] Stream ended
Interrupted! Exiting...
[cli][info] Closing currently open stream...
this is de info Url is a paid service with my personal info...
streamlink --loglevel=debug --output=/dev/null --ffmpeg-ffmpeg=/usr/bin/ffmpeg "XXX.m3u8"
ffmpeg -version 2>/dev/null | head -n1
ffmpeg version 5.1.1-static https://johnvansickle.com/ffmpeg/ Copyright (c) 2000-2022 the FFmpeg developers
This looks like an issue with your ffmpeg binary and not with Streamlink. As I've shown in the link above, Streamlink reads the first line of the stdout stream and tries to match it with the version string regex, which is `^ffmpeg version (\S+)`.
In order for the ffmpeg binary to be considered valid, it must exit with status code 0 and the version string must have matched.
https://github.com/streamlink/streamlink/blob/6.0.0/src/streamlink/stream/ffmpegmux.py#L274-L286
Post the full stdout and stderr output when running ffmpeg (using the absolute path)
```bash
/usr/bin/ffmpeg -version
```
and post its status/exit code
```bash
echo $?
```
----
There's also `--ffmpeg-no-validation` for skipping the validation step:
https://streamlink.github.io/cli.html#cmdoption-ffmpeg-no-validation
/usr/bin/ffmpeg -version
ffmpeg version 5.1.1-static https://johnvansickle.com/ffmpeg/ Copyright (c) 2000-2022 the FFmpeg developers
#echo $?
0
i tried --ffmpeg-no-validation and its now working.
Thank you for the help.
> /usr/bin/ffmpeg -version
> ffmpeg version 5.1.1-static [johnvansickle.com/ffmpeg](https://johnvansickle.com/ffmpeg/) Copyright (c) 2000-2022 the FFmpeg developers
There is no way that `-version` doesn't also output the ffmpeg build options. You're either leaving this out, or something else is going on here. I have checked the 5.1.1 build and 6.0 build from that site in my docker container and both behave as I'm expecting.
Please check the FFmpeg builds from here as well, because `--ffmpeg-no-validation` is not a solution:
https://github.com/BtbN/FFmpeg-Builds
----
It's possible that there is an async issue with the spawned child process in python where it processes the termination task first before processing the stdout task, hence why it doesn't validate an exit code of 0. If your ffmpeg binary only spits out one line, then this could explain the async timing issue.
https://github.com/streamlink/streamlink/blob/6.0.0/src/streamlink/utils/processoutput.py#L32
I will have a look at rewriting the `ProcessOutput` class using `trio` at some point though because the code would be much cleaner. This could fix a potential issue like this.
> Please check the FFmpeg builds from here as well, because `--ffmpeg-no-validation` is not a solution: https://github.com/BtbN/FFmpeg-Builds
I have checked the ffmpeg build.
I think my VPS is to old.
On my other local (@ home) linux system it works fine. No --ffmpeg-no-validation. That system is newer. Unfortunately i can at the moment not upgrade my VPS.
The nightly ffmpeg build works also fine on my local system.
Thank you.
i have met this issue too , don't ask me what static build I was using I don't remember.
You've removed parts of the debug log, so it's unclear which ffmpeg binary you've actually set.
Streamlink checks the first line of the stdout stream when running `ffmpeg -version`, as you can see here:
https://github.com/streamlink/streamlink/blob/6.0.0/src/streamlink/stream/ffmpegmux.py#L261-L267
The output of these custom-built ffmpeg binaries works just fine:
```
$ ./ffmpeg-6.0-amd64-static/ffmpeg -version 2>/dev/null | head -n1
ffmpeg version 6.0-static https://johnvansickle.com/ffmpeg/ Copyright (c) 2000-2023 the FFmpeg developers
```
Output from a temp docker container running the ffmpeg binary:
```
$ /opt/python/cp311-cp311/bin/python -m streamlink --loglevel=debug --output=/dev/null --ffmpeg-ffmpeg=/ffmpeg/ffmpeg-6.0-amd64-static/ffmpeg https://steamcommunity.com/broadcast/watch/76561199514732246 best
[cli][info] streamlink is running as root! Be careful!
[cli][debug] OS: Linux-6.4.6-1-git-x86_64-with-glibc2.17
[cli][debug] Python: 3.11.4
[cli][debug] Streamlink: 6.0.0
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.7.22
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.3
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.18.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.22.2
[cli][debug] trio-websocket: 0.10.3
[cli][debug] typing-extensions: 4.7.1
[cli][debug] urllib3: 2.0.4
[cli][debug] websocket-client: 1.6.1
[cli][debug] Arguments:
[cli][debug] url=https://steamcommunity.com/broadcast/watch/76561199514732246
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --output=/dev/null
[cli][debug] --ffmpeg-ffmpeg=/ffmpeg/ffmpeg-6.0-amd64-static/ffmpeg
[cli][info] Found matching plugin steam for URL https://steamcommunity.com/broadcast/watch/76561199514732246
[plugins.steam][debug] Getting broadcast stream: sessionid=d3e4ef22f1a58e991fc13ab4
[utils.l10n][debug] Language code: en_US
[stream.dash][debug] Available languages for DASH audio streams: und (using: und)
[cli][info] Available streams: 360p (worst), 480p, 720p, 1080p (best)
[cli][info] Opening stream: 1080p (dash)
[cli][info] Writing output to
/dev/null
[cli][debug] Checking file output
[stream.dash][debug] Opening DASH reader for: ('1', 'video', '1') - video/mp4
[stream.dash][debug] Opening DASH reader for: ('1', 'audio', '1') - audio/mp4
[stream.dash][debug] video/mp4 segment initialization: downloading (2023-07-25T22:09:33.000000Z / 2023-07-27T17:25:15.525464Z)
[stream.dash_manifest][debug] Generating segment numbers for dynamic playlist: ('1', 'video', '1')
[stream.dash_manifest][debug] Stream start: 2023-07-25 22:09:33+00:00
[stream.dash_manifest][debug] Current time: 2023-07-27 17:25:15.523858+00:00
[stream.dash_manifest][debug] Availability: 2023-07-27 17:25:09+00:00
[stream.dash_manifest][debug] presentationTimeOffset: 0:00:00; suggestedPresentationDelay: 0:00:03; minBufferTime: 0:00:03
[stream.dash_manifest][debug] segmentDuration: 2.0; segmentStart: 1; segmentOffset: 77868 (155736.523858s)
[stream.dash][debug] audio/mp4 segment initialization: downloading (2023-07-25T22:09:33.000000Z / 2023-07-27T17:25:15.528351Z)
[stream.dash_manifest][debug] Generating segment numbers for dynamic playlist: ('1', 'audio', '1')
[stream.dash_manifest][debug] Stream start: 2023-07-25 22:09:33+00:00
[stream.dash_manifest][debug] Current time: 2023-07-27 17:25:15.523858+00:00
[stream.dash_manifest][debug] Availability: 2023-07-27 17:25:09+00:00
[stream.dash_manifest][debug] presentationTimeOffset: 0:00:00; suggestedPresentationDelay: 0:00:03; minBufferTime: 0:00:03
[stream.dash_manifest][debug] segmentDuration: 2.0; segmentStart: 1; segmentOffset: 77868 (155736.523858s)
[stream.ffmpegmux][debug] ffmpeg version 6.0-static https://johnvansickle.com/ffmpeg/ Copyright (c) 2000-2023 the FFmpeg developers
[stream.ffmpegmux][debug] built with gcc 8 (Debian 8.3.0-6)
[stream.ffmpegmux][debug] configuration: --enable-gpl --enable-version3 --enable-static --disable-debug --disable-ffplay --disable-indev=sndio --disable-outdev=sndio --cc=gcc --enable-fontconfig --enable-frei0r --enable-gnutls --enable-gmp --enable-libgme --enable-gray --enable-libaom --enable-libfribidi --enable-libass --enable-libvmaf --enable-libfreetype --enable-libmp3lame --enable-libopencore-amrnb --enable-libopencore-amrwb --enable-libopenjpeg --enable-librubberband --enable-libsoxr --enable-libspeex --enable-libsrt --enable-libvorbis --enable-libopus --enable-libtheora --enable-libvidstab --enable-libvo-amrwbenc --enable-libvpx --enable-libwebp --enable-libx264 --enable-libx265 --enable-libxml2 --enable-libdav1d --enable-libxvid --enable-libzvbi --enable-libzimg
[stream.ffmpegmux][debug] libavutil 58. 2.100 / 58. 2.100
[stream.ffmpegmux][debug] libavcodec 60. 3.100 / 60. 3.100
[stream.ffmpegmux][debug] libavformat 60. 3.100 / 60. 3.100
[stream.ffmpegmux][debug] libavdevice 60. 1.100 / 60. 1.100
[stream.ffmpegmux][debug] libavfilter 9. 3.100 / 9. 3.100
[stream.ffmpegmux][debug] libswscale 7. 1.100 / 7. 1.100
[stream.ffmpegmux][debug] libswresample 4. 10.100 / 4. 10.100
[stream.ffmpegmux][debug] libpostproc 57. 1.100 / 57. 1.100
[utils.named_pipe][info] Creating pipe streamlinkpipe-53-1-9459
[utils.named_pipe][info] Creating pipe streamlinkpipe-53-2-4204
[stream.ffmpegmux][debug] ffmpeg command: /ffmpeg/ffmpeg-6.0-amd64-static/ffmpeg -nostats -y -i /tmp/streamlinkpipe-53-1-9459 -i /tmp/streamlinkpipe-53-2-4204 -c:v copy -c:a copy -copyts -f matroska pipe:1
[stream.ffmpegmux][debug] Starting copy to pipe: /tmp/streamlinkpipe-53-1-9459
[stream.ffmpegmux][debug] Starting copy to pipe: /tmp/streamlinkpipe-53-2-4204
[cli][debug] Pre-buffering 8192 bytes
[stream.dash][debug] audio/mp4 segment 77869: downloading (2023-07-27T17:25:09.000000Z / 2023-07-27T17:25:15.653844Z)
[stream.dash][debug] audio/mp4 segment initialization: completed
[stream.dash][debug] audio/mp4 segment 77870: downloading (2023-07-27T17:25:11.000000Z / 2023-07-27T17:25:15.695339Z)
[stream.dash][debug] audio/mp4 segment 77869: completed
[stream.dash][debug] audio/mp4 segment 77871: downloading (2023-07-27T17:25:13.000000Z / 2023-07-27T17:25:15.755956Z)
[stream.dash][debug] audio/mp4 segment 77870: completed
[stream.dash][debug] video/mp4 segment 77869: downloading (2023-07-27T17:25:09.000000Z / 2023-07-27T17:25:15.783191Z)
[stream.dash][debug] video/mp4 segment initialization: completed
[stream.dash][debug] audio/mp4 segment 77872: downloading (2023-07-27T17:25:15.000000Z / 2023-07-27T17:25:15.808912Z)
[stream.dash][debug] audio/mp4 segment 77871: completed
[stream.dash][debug] audio/mp4 segment 77873: waiting 1.1s (2023-07-27T17:25:17.000000Z / 2023-07-27T17:25:15.896141Z)
[stream.dash][debug] audio/mp4 segment 77872: completed
[stream.dash][debug] video/mp4 segment 77870: downloading (2023-07-27T17:25:11.000000Z / 2023-07-27T17:25:16.888916Z)
[stream.dash][debug] video/mp4 segment 77869: completed
[stream.dash][debug] audio/mp4 segment 77873: downloading (2023-07-27T17:25:17.000000Z / 2023-07-27T17:25:17.000241Z)
[cli][debug] Writing stream to output
[stream.dash][debug] audio/mp4 segment 77874: waiting 1.8s (2023-07-27T17:25:19.000000Z / 2023-07-27T17:25:17.200659Z)
[stream.dash][debug] audio/mp4 segment 77873: completed
[download] Written 592.00 KiB to /dev/null (0s) [stream.dash][debug] video/mp4 segment 77871: downloading (2023-07-27T17:25:13.000000Z / 2023-07-27T17:25:17.302594Z)
[stream.dash][debug] video/mp4 segment 77870: completed
^C
[stream.ffmpegmux][debug] Closing ffmpeg thread
[stream.segmented][debug] Closing worker thread
[stream.segmented][debug] Closing writer thread
[stream.ffmpegmux][debug] Pipe copy complete: /tmp/streamlinkpipe-53-1-9459
[stream.segmented][debug] Closing worker thread
[stream.segmented][debug] Closing writer thread
[stream.ffmpegmux][debug] Pipe copy complete: /tmp/streamlinkpipe-53-2-4204
[stream.dash][debug] audio/mp4 segment 77874: cancelled
[stream.dash][warning] video/mp4 segment 77871: aborted
[stream.ffmpegmux][debug] Closed all the substreams
[cli][info] Stream ended
Interrupted! Exiting...
[cli][info] Closing currently open stream...
```
[cli][debug] OS: Linux-3.10.0-1062.4.1.el7.x86_64-x86_64-with-glibc2.17
[cli][debug] Python: 3.11.4
[cli][debug] Streamlink: 6.0.0
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.5.7
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.2
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.18.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.22.2
[cli][debug] trio-websocket: 0.10.3
[cli][debug] typing-extensions: 4.7.1
[cli][debug] urllib3: 2.0.3
[cli][debug] websocket-client: 1.6.1
[cli][debug] Arguments:
[cli][debug] url=XXX
[cli][debug] --loglevel=debug
[cli][debug] --player=mpv
[cli][debug] --player-no-close=True
[cli][debug] --output=/dev/null
[cli][debug] --default-stream=['720p', 'best', 'vod']
[cli][debug] --ringbuffer-size=33554432
[cli][debug] --stream-segment-attempts=4
[cli][debug] --stream-segment-timeout=30.0
[cli][debug] --stream-timeout=60.0
[cli][debug] --hls-playlist-reload-attempts=4
[cli][debug] --hls-playlist-reload-time=segment
[cli][debug] --ffmpeg-ffmpeg=/usr/bin/ffmpeg
[cli][debug] --http-header=[('User-Agent', '"Mozilla/5.0 (Windows NT 6.1; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/75.0.3770.100 Safari/537.36 OPR/62.0.3331.66"'), ('User-Agent', 'Mozilla/5.0 (Windows NT 10.0; rv:114.0) Gecko/20100101 Firefox/114.0')]
[cli][debug] --twitch-disable-ads=True
[cli][debug] --twitch-disable-hosting=True
[cli][info] Found matching plugin hls for URL XXX
[plugins.hls][debug] URL=private
[utils.l10n][debug] Language code: en_US
[stream.ffmpegmux][error] Could not validate FFmpeg!
[stream.ffmpegmux][error] Unexpected FFmpeg version output while running ['/usr/bin/ffmpeg', '-version']
[stream.ffmpegmux][warning] No valid FFmpeg binary was found. See the --ffmpeg-ffmpeg option.
[stream.ffmpegmux][warning] Muxing streams is unsupported! Only a subset of the available streams can be returned!
[cli][info] Available streams: 234p_alt (worst), 234p, 360p_alt2, 360p_alt, 360p, 540p, 720p_alt, 720p, 1080p (best)
[cli][info] Opening stream: 720p (hls)
[cli][info] Writing output to
/dev/null
[cli][debug] Checking file output
[stream.hls][debug] Reloading playlist
[cli][debug] Pre-buffering 8192 bytes
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] First Sequence: 4946541; Last Sequence: 4947172
[stream.hls][debug] Start offset: 0; Duration: None; Start Sequence: 4947170; End Sequence: None
[stream.hls][debug] Adding segment 4947170 to queue
[stream.hls][debug] Adding segment 4947171 to queue
[stream.hls][debug] Adding segment 4947172 to queue
[stream.hls][debug] Writing segment 4947170 to output
[stream.hls][debug] Segment 4947170 complete
[stream.hls][debug] Writing segment 4947171 to output
[stream.hls][debug] Segment 4947171 complete
[stream.hls][debug] Writing segment 4947172 to output
[stream.hls][debug] Segment 4947172 complete
[cli][debug] Writing stream to output
[download] Written 1.36 MiB to /dev/null (4s @ 303.87 KiB/s)
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 4947173 to queue
[stream.hls][debug] Writing segment 4947173 to output
[stream.hls][debug] Segment 4947173 complete
[download] Written 1.83 MiB to /dev/null (6s @ 292.90 KiB/s)
^C
[stream.segmented][debug] Closing worker thread
[stream.segmented][debug] Closing writer thread
[cli][info] Stream ended
Interrupted! Exiting...
[cli][info] Closing currently open stream...
this is de info Url is a paid service with my personal info...
streamlink --loglevel=debug --output=/dev/null --ffmpeg-ffmpeg=/usr/bin/ffmpeg "XXX.m3u8"
ffmpeg -version 2>/dev/null | head -n1
ffmpeg version 5.1.1-static https://johnvansickle.com/ffmpeg/ Copyright (c) 2000-2022 the FFmpeg developers
This looks like an issue with your ffmpeg binary and not with Streamlink. As I've shown in the link above, Streamlink reads the first line of the stdout stream and tries to match it with the version string regex, which is `^ffmpeg version (\S+)`.
In order for the ffmpeg binary to be considered valid, it must exit with status code 0 and the version string must have matched.
https://github.com/streamlink/streamlink/blob/6.0.0/src/streamlink/stream/ffmpegmux.py#L274-L286
Post the full stdout and stderr output when running ffmpeg (using the absolute path)
```bash
/usr/bin/ffmpeg -version
```
and post its status/exit code
```bash
echo $?
```
----
There's also `--ffmpeg-no-validation` for skipping the validation step:
https://streamlink.github.io/cli.html#cmdoption-ffmpeg-no-validation
/usr/bin/ffmpeg -version
ffmpeg version 5.1.1-static https://johnvansickle.com/ffmpeg/ Copyright (c) 2000-2022 the FFmpeg developers
#echo $?
0
i tried --ffmpeg-no-validation and its now working.
Thank you for the help.
> /usr/bin/ffmpeg -version
> ffmpeg version 5.1.1-static [johnvansickle.com/ffmpeg](https://johnvansickle.com/ffmpeg/) Copyright (c) 2000-2022 the FFmpeg developers
There is no way that `-version` doesn't also output the ffmpeg build options. You're either leaving this out, or something else is going on here. I have checked the 5.1.1 build and 6.0 build from that site in my docker container and both behave as I'm expecting.
Please check the FFmpeg builds from here as well, because `--ffmpeg-no-validation` is not a solution:
https://github.com/BtbN/FFmpeg-Builds
----
It's possible that there is an async issue with the spawned child process in python where it processes the termination task first before processing the stdout task, hence why it doesn't validate an exit code of 0. If your ffmpeg binary only spits out one line, then this could explain the async timing issue.
https://github.com/streamlink/streamlink/blob/6.0.0/src/streamlink/utils/processoutput.py#L32
I will have a look at rewriting the `ProcessOutput` class using `trio` at some point though because the code would be much cleaner. This could fix a potential issue like this.
> Please check the FFmpeg builds from here as well, because `--ffmpeg-no-validation` is not a solution: https://github.com/BtbN/FFmpeg-Builds
I have checked the ffmpeg build.
I think my VPS is to old.
On my other local (@ home) linux system it works fine. No --ffmpeg-no-validation. That system is newer. Unfortunately i can at the moment not upgrade my VPS.
The nightly ffmpeg build works also fine on my local system.
Thank you.
i have met this issue too , don't ask me what static build I was using I don't remember. | 2023-08-04T22:29:31 |
streamlink/streamlink | 5,484 | streamlink__streamlink-5484 | [
"5481"
] | 3a38ca2e6f66a6b11ea4d96b8d0f0f9c780f9948 | diff --git a/src/streamlink_cli/argparser.py b/src/streamlink_cli/argparser.py
--- a/src/streamlink_cli/argparser.py
+++ b/src/streamlink_cli/argparser.py
@@ -15,21 +15,13 @@
from streamlink_cli.utils import find_default_player
-_printable_re = re.compile(r"[{0}]".format(printable))
-_option_re = re.compile(r"""
- (?P<name>[A-z-]+) # A option name, valid characters are A to z and dash.
- \s*
- (?P<op>=)? # Separating the option and the value with a equals sign is
- # common, but optional.
- \s*
- (?P<value>.*) # The value, anything goes.
-""", re.VERBOSE)
-
-
class ArgumentParser(argparse.ArgumentParser):
# noinspection PyUnresolvedReferences,PyProtectedMember
NESTED_ARGUMENT_GROUPS: Dict[Optional[argparse._ArgumentGroup], List[argparse._ArgumentGroup]]
+ _RE_PRINTABLE = re.compile(fr"[{re.escape(printable)}]")
+ _RE_OPTION = re.compile(r"^(?P<name>[A-Za-z0-9-]+)(?:(?P<op>\s*=\s*|\s+)(?P<value>.*))?$")
+
def __init__(self, *args, **kwargs):
self.NESTED_ARGUMENT_GROUPS = {}
super().__init__(*args, **kwargs)
@@ -51,21 +43,22 @@ def add_argument_group(
def convert_arg_line_to_args(self, line):
# Strip any non-printable characters that might be in the
# beginning of the line (e.g. Unicode BOM marker).
- match = _printable_re.search(line)
+ match = self._RE_PRINTABLE.search(line)
if not match:
return
line = line[match.start():].strip()
# Skip lines that do not start with a valid option (e.g. comments)
- option = _option_re.match(line)
+ option = self._RE_OPTION.match(line)
if not option:
return
- name, value = option.group("name", "value")
- if name and value:
- yield f"--{name}={value}"
- elif name:
- yield f"--{name}"
+ name, op, value = option.group("name", "op", "value")
+ prefix = self.prefix_chars[0] if len(name) == 1 else self.prefix_chars[0] * 2
+ if value or op:
+ yield f"{prefix}{name}={value}"
+ else:
+ yield f"{prefix}{name}"
def _match_argument(self, action, arg_strings_pattern):
# - https://github.com/streamlink/streamlink/issues/971
| diff --git a/tests/cli/test_argparser.py b/tests/cli/test_argparser.py
--- a/tests/cli/test_argparser.py
+++ b/tests/cli/test_argparser.py
@@ -1,4 +1,6 @@
+import argparse
from argparse import ArgumentParser, Namespace
+from pathlib import Path
from typing import Any, List
from unittest.mock import Mock
@@ -14,6 +16,65 @@ def parser():
return build_parser()
+class TestConfigFileArguments:
+ @pytest.fixture()
+ def parsed(self, request: pytest.FixtureRequest, parser: argparse.ArgumentParser, tmp_path: Path):
+ content = "\n".join([
+ "",
+ " ",
+ "# comment",
+ "! comment",
+ "invalid_option_format",
+ *getattr(request, "param", []),
+ ])
+
+ config = tmp_path / "config"
+ with config.open("w") as fd:
+ fd.write(content)
+
+ return parser.parse_args([f"@{config}"])
+
+ @pytest.mark.parametrize("parsed", [[]], indirect=True)
+ def test_nooptions(self, parsed: Namespace):
+ assert parsed.ipv4 is None
+ assert parsed.player_fifo is False
+ assert parsed.player_args == ""
+ assert parsed.title is None
+
+ @pytest.mark.parametrize("parsed", [
+ pytest.param(["4"], id="shorthand name"),
+ pytest.param(["ipv4"], id="full name"),
+ ], indirect=True)
+ def test_alphanumerical(self, parsed: Namespace):
+ assert parsed.ipv4 is True
+
+ @pytest.mark.parametrize("parsed", [
+ pytest.param(["n"], id="shorthand name"),
+ pytest.param(["player-fifo"], id="full name"),
+ ], indirect=True)
+ def test_withoutvalue(self, parsed: Namespace):
+ assert parsed.player_fifo is True
+
+ @pytest.mark.parametrize("parsed", [
+ pytest.param(["a=foo bar "], id="shorthand name with operator"),
+ pytest.param(["a = foo bar "], id="shorthand name with operator and surrounding whitespace"),
+ pytest.param(["a foo bar "], id="shorthand name without operator"),
+ pytest.param(["player-args=foo bar "], id="full name with operator"),
+ pytest.param(["player-args = foo bar "], id="full name with operator and surrounding whitespace"),
+ pytest.param(["player-args foo bar "], id="full name without operator"),
+ ], indirect=True)
+ def test_withvalue(self, parsed: Namespace):
+ assert parsed.player_args == "foo bar"
+
+ @pytest.mark.parametrize("parsed", [
+ pytest.param(["title="], id="operator"),
+ pytest.param(["title ="], id="operator with leading whitespace"),
+ pytest.param(["title = "], id="operator with surrounding whitespace"),
+ ], indirect=True)
+ def test_emptyvalue(self, parsed: Namespace):
+ assert parsed.title == ""
+
+
@pytest.mark.filterwarnings("ignore")
@pytest.mark.parametrize(("argv", "option", "expected"), [
pytest.param(
| ipv4 setting in config file doesn't work
### Checklist
- [X] This is a bug report and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed bug reports](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22bug%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest stable release
### Description
With `ipv4` in the config file, streamlink --loglevel=debug $URL produces:
```
usage: streamlink [OPTIONS] <URL> [STREAM]
streamlink: error: ambiguous option: --ipv=4 could match --ipv4, --ipv6
```
With just `4`, it works
### Debug log
```text
streamlink --loglevel=debug <url>
usage: streamlink [OPTIONS] <URL> [STREAM]
streamlink: error: ambiguous option: --ipv=4 could match --ipv4, --ipv6
```
| Thanks. Looks like it's caused by this poor regex:
- https://github.com/streamlink/streamlink/blame/6.0.1/src/streamlink_cli/argparser.py#L19-L26
- https://github.com/streamlink/streamlink/blame/6.0.1/src/streamlink_cli/argparser.py#L59-L62
Tests were never added to this `argparse.ArgumentParser` override:
https://app.codecov.io/gh/streamlink/streamlink/commit/4a234ff76a73ad238eea8470267366592963ad50/blob/src/streamlink_cli/argparser.py#L51
Going to fix this later today...
See #5484 | 2023-08-05T13:24:07 |
streamlink/streamlink | 5,504 | streamlink__streamlink-5504 | [
"5498"
] | 130238289ed0aeeaa341101bd00ab92e3536332c | diff --git a/src/streamlink/stream/dash.py b/src/streamlink/stream/dash.py
--- a/src/streamlink/stream/dash.py
+++ b/src/streamlink/stream/dash.py
@@ -26,8 +26,8 @@ class DASHStreamWriter(SegmentedStreamWriter):
reader: "DASHStreamReader"
stream: "DASHStream"
- def fetch(self, segment: Segment, retries: Optional[int] = None):
- if self.closed or not retries:
+ def fetch(self, segment: Segment):
+ if self.closed:
return
name = segment.name
@@ -53,7 +53,7 @@ def fetch(self, segment: Segment, retries: Optional[int] = None):
timeout=self.timeout,
exception=StreamError,
headers=headers,
- retries=retries,
+ retries=self.retries,
**request_args,
)
except StreamError as err:
@@ -175,10 +175,8 @@ def __init__(
stream: "DASHStream",
representation: Representation,
timestamp: datetime,
- *args,
- **kwargs,
):
- super().__init__(stream, *args, **kwargs)
+ super().__init__(stream)
self.ident = representation.ident
self.mime_type = representation.mimeType
self.timestamp = timestamp
diff --git a/src/streamlink/stream/segmented.py b/src/streamlink/stream/segmented.py
--- a/src/streamlink/stream/segmented.py
+++ b/src/streamlink/stream/segmented.py
@@ -50,59 +50,10 @@ def wait(self, time: float) -> bool:
return not self._wait.wait(time)
-class SegmentedStreamWorker(AwaitableMixin, Thread):
- """The general worker thread.
-
- This thread is responsible for queueing up segments in the
- writer thread.
- """
-
- reader: "SegmentedStreamReader"
- writer: "SegmentedStreamWriter"
- stream: "Stream"
-
- def __init__(self, reader: "SegmentedStreamReader", **kwargs):
- super().__init__(daemon=True, name=f"Thread-{self.__class__.__name__}")
- self.closed = False
- self.reader = reader
- self.writer = reader.writer
- self.stream = reader.stream
- self.session = reader.session
-
- def close(self):
- """Shuts down the thread."""
- if self.closed: # pragma: no cover
- return
-
- log.debug("Closing worker thread")
-
- self.closed = True
- self._wait.set()
-
- def iter_segments(self):
- """The iterator that generates segments for the worker thread.
-
- Should be overridden by the inheriting class.
- """
- return
- yield
-
- def run(self):
- for segment in self.iter_segments():
- if self.closed: # pragma: no cover
- break
- self.writer.put(segment)
-
- # End of stream, tells the writer to exit
- self.writer.put(None)
- self.close()
-
-
class SegmentedStreamWriter(AwaitableMixin, Thread):
- """The writer thread.
-
- This thread is responsible for fetching segments, processing them
- and finally writing the data to the buffer.
+ """
+ The base writer thread.
+ This thread is responsible for fetching segments, processing them and finally writing the data to the buffer.
"""
reader: "SegmentedStreamReader"
@@ -110,28 +61,25 @@ class SegmentedStreamWriter(AwaitableMixin, Thread):
def __init__(self, reader: "SegmentedStreamReader", size=20, retries=None, threads=None, timeout=None):
super().__init__(daemon=True, name=f"Thread-{self.__class__.__name__}")
+
self.closed = False
+
self.reader = reader
self.stream = reader.stream
self.session = reader.session
- if not retries:
- retries = self.session.options.get("stream-segment-attempts")
+ self.retries = retries or self.session.options.get("stream-segment-attempts")
+ self.threads = threads or self.session.options.get("stream-segment-threads")
+ self.timeout = timeout or self.session.options.get("stream-segment-timeout")
- if not threads:
- threads = self.session.options.get("stream-segment-threads")
-
- if not timeout:
- timeout = self.session.options.get("stream-segment-timeout")
-
- self.retries = retries
- self.timeout = timeout
- self.threads = threads
self.executor = CompatThreadPoolExecutor(max_workers=self.threads)
- self.futures: queue.Queue[Future] = queue.Queue(size)
+ self._queue: queue.Queue[Future] = queue.Queue(size)
def close(self):
- """Shuts down the thread, its executor and closes the reader (worker thread and buffer)."""
+ """
+ Shuts down the thread, its executor and closes the reader (worker thread and buffer).
+ """
+
if self.closed: # pragma: no cover
return
@@ -144,59 +92,67 @@ def close(self):
self.executor.shutdown(wait=True, cancel_futures=True)
def put(self, segment):
- """Adds a segment to the download pool and write queue."""
+ """
+ Adds a segment to the download pool and write queue.
+ """
+
if self.closed: # pragma: no cover
return
if segment is None:
future = None
else:
- future = self.executor.submit(self.fetch, segment, retries=self.retries)
+ future = self.executor.submit(self.fetch, segment)
self.queue(segment, future)
def queue(self, segment: Any, future: Optional[Future], *data):
- """Puts values into a queue but aborts if this thread is closed."""
+ """
+ Puts values into a queue but aborts if this thread is closed.
+ """
+
+ item = None if segment is None or future is None else (segment, future, data)
while not self.closed: # pragma: no branch
try:
- self._futures_put((segment, future, *data))
+ self._queue_put(item)
return
except queue.Full: # pragma: no cover
continue
- def _futures_put(self, item):
- self.futures.put(item, block=True, timeout=1)
+ def _queue_put(self, item):
+ self._queue.put(item, block=True, timeout=1)
- def _futures_get(self):
- return self.futures.get(block=True, timeout=0.5)
+ def _queue_get(self):
+ return self._queue.get(block=True, timeout=0.5)
@staticmethod
def _future_result(future: Future):
return future.result(timeout=0.5)
def fetch(self, segment):
- """Fetches a segment.
-
+ """
+ Fetches a segment.
Should be overridden by the inheriting class.
"""
def write(self, segment, result, *data):
- """Writes a segment to the buffer.
-
+ """
+ Writes a segment to the buffer.
Should be overridden by the inheriting class.
"""
def run(self):
while not self.closed:
try:
- segment, future, *data = self._futures_get()
+ item = self._queue_get()
except queue.Empty: # pragma: no cover
continue
# End of stream
- if future is None:
+ if item is None:
break
+ segment, future, data = item
while not self.closed: # pragma: no branch
try:
result = self._future_result(future)
@@ -213,6 +169,60 @@ def run(self):
self.close()
+class SegmentedStreamWorker(AwaitableMixin, Thread):
+ """
+ The base worker thread.
+ This thread is responsible for queueing up segments in the writer thread.
+ """
+
+ reader: "SegmentedStreamReader"
+ writer: "SegmentedStreamWriter"
+ stream: "Stream"
+
+ def __init__(self, reader: "SegmentedStreamReader", **kwargs):
+ super().__init__(daemon=True, name=f"Thread-{self.__class__.__name__}")
+
+ self.closed = False
+
+ self.reader = reader
+ self.writer = reader.writer
+ self.stream = reader.stream
+ self.session = reader.session
+
+ def close(self):
+ """
+ Shuts down the thread.
+ """
+
+ if self.closed: # pragma: no cover
+ return
+
+ log.debug("Closing worker thread")
+
+ self.closed = True
+ self._wait.set()
+
+ def iter_segments(self):
+ """
+ The iterator that generates segments for the worker thread.
+ Should be overridden by the inheriting class.
+ """
+
+ return
+ # noinspection PyUnreachableCode
+ yield
+
+ def run(self):
+ for segment in self.iter_segments():
+ if self.closed: # pragma: no cover
+ break
+ self.writer.put(segment)
+
+ # End of stream, tells the writer to exit
+ self.writer.put(None)
+ self.close()
+
+
class SegmentedStreamReader(StreamIO):
__worker__ = SegmentedStreamWorker
__writer__ = SegmentedStreamWriter
@@ -221,14 +231,17 @@ class SegmentedStreamReader(StreamIO):
writer: "SegmentedStreamWriter"
stream: "Stream"
- def __init__(self, stream: "Stream", timeout=None):
+ def __init__(self, stream: "Stream"):
super().__init__()
+
self.stream = stream
self.session = stream.session
- self.timeout = timeout or self.session.options.get("stream-timeout")
+
+ self.timeout = self.session.options.get("stream-timeout")
buffer_size = self.session.get_option("ringbuffer-size")
self.buffer = RingBuffer(buffer_size)
+
self.writer = self.__writer__(self)
self.worker = self.__worker__(self)
| diff --git a/tests/mixins/stream_hls.py b/tests/mixins/stream_hls.py
--- a/tests/mixins/stream_hls.py
+++ b/tests/mixins/stream_hls.py
@@ -114,11 +114,11 @@ def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.handshake = Handshake()
- def _futures_put(self, item):
- self.futures.put_nowait(item)
+ def _queue_put(self, item):
+ self._queue.put_nowait(item)
- def _futures_get(self):
- return self.futures.get_nowait()
+ def _queue_get(self):
+ return self._queue.get_nowait()
@staticmethod
def _future_result(future):
diff --git a/tests/stream/test_hls.py b/tests/stream/test_hls.py
--- a/tests/stream/test_hls.py
+++ b/tests/stream/test_hls.py
@@ -774,11 +774,11 @@ def mocked_playlist_reload_time(*args, **kwargs):
return orig_playlist_reload_time(*args, **kwargs)
# immediately kill the writer thread as we don't need it and don't want to wait for its queue polling to end
- def mocked_futures_get():
- return None, None
+ def mocked_queue_get():
+ return None
with patch.object(thread.reader.worker, "_playlist_reload_time", side_effect=mocked_playlist_reload_time), \
- patch.object(thread.reader.writer, "_futures_get", side_effect=mocked_futures_get):
+ patch.object(thread.reader.writer, "_queue_get", side_effect=mocked_queue_get):
self.start()
if not playlist_reload_time_called.wait(timeout=5): # pragma: no cover
| Refactor segmented streams and use a common BaseSegment
While talking about the extensibility and lack of full typing information of the segmented stream implementations in the past, I already mentioned that the base implementations of segmented streams, as well as HLS and DASH are in need of a huge code refactoring.
Now with my attempt at turning the `--hls-duration` and `--hls-start-offset` options into the generic `--stream-segmented-duration` and `--stream-segmented-start-offset` options, it became clear to me that it didn't make sense finishing that work with the current code.
See #5450 and https://github.com/streamlink/streamlink/pull/5493#issuecomment-1677680058.
----
The issue I'm intending to solve, ignoring all other flaws for now (there are a lot), is making both HLS, DASH and other subclasses of the segmented stream base classes share more common logic in regards to how segments are handled. I also want to fix the lack of typing information, so working with and understanding the segmented stream implementations is much easier.
Currently, each segmented stream implementation does its own thing with its segments, so implementing common logic for calculating a max stream duration or a stream start offset for example is impossible without writing lots of duplicate code with only small variations. This is also the reason why adding proper typing information can't be done. Writing logic for the same thing in each segmented stream type just doesn't make sense.
----
The big outlier here is HLS, where segments are wrapped in a `Sequence` named tuple. This is due to historic reasons when the segmented streams were initially implemented. Segments of each stream type were defined as named tuples which don't support subclassing or changing values after instantiation, so in order for the HLS stream's M3U8 parser to work properly, the generated segments needed to be wrapped, so a sequence number could be added after parsing the playlist as a whole (`EXT-X-MEDIA-SEQUENCE`).
While refactoring/rewriting the DASH implementation earlier this year, I already replaced its segments with ones that are based on the stdlib's `dataclasses`. Those fix all the issues that come with named tuples, but `dataclasses` come at the cost of a slight performance hit compared to named tuples. That is negligible though and is not important. It doesn't matter for Streamlink.
With dataclass segments, a `BaseSegment` type can be created which HLS, DASH and other can inherit from. The base `SegmentedStream{Worker,Writer,Reader}` classes can then properly handle the `BaseSegment` objects, and common base logic for max stream durations and start offsets could be added. Then fixing the lack of typing information would be much easier, too.
----
While this would affect huge parts of the base segmented stream stuff, as well as HLS and DASH, I don't think that there are any breaking changes involved. Public interfaces have never been declared on the stream implementations other than the main classes' constructors and classmethods like `HLSStream.parse_variant_stream()` and `DASHStream.parse_manifest()`. Those won't be touched.
Anything internal, like certain attribute names and methods, regardless whether they have docstrings attached or their names not starting with an underscore character are not part of the public interface, even if some plugins create stream subclasses and override them.
That's why I won't feel bad if any changes being made will break 3rd party plugins.
----
My initial improvised plan is this:
- [x] Refactor `stream.segmented`
- [x] Change how the segments get put into and read from the queue (#5504)
- [x] Add typing, with generic classes which define the segment type and segment data return type (#5507)
- [x] Refactor `stream.hls` and `stream.hls_playlist` (#5526)
- Turn `Segment` and `Playlist` from named tuples into dataclasses
- Move segment number generation from `HLSStreamWorker` to the `M3U8` parser
- Replace the `Sequence` named tuple wrapper in HLS streams with a flat `Segment` dataclass type
- Rename internal `HLSStream{Writer,Worker}` attributes and methods (including args+keywords)
- Refactor M3U8 playlist loading
- Fix / add more typing annotations
- [x] Refactor `stream.dash` and `stream.dash_manifest`
- Fix/update all the worker/writer/reader parts
- Fix / add more typing
- [x] Refactor / fix plugins
- [x] `plugins.twitch` (#5526)
- [x] `plugins.ustreamtv`
- [x] other plugins with HLS stream subclasses (#5526)
- [x] Switch to sub-packages for `stream.segmented`, `stream.hls` and `stream.dash`
- [x] Rename HLS `Segment` to `HLSSegment` and DASH `Segment` to `DASHSegment`
- [x] Add base `Segment` with common attributes (`uri`, `num`, `duration`)
- [x] Make `{HLS,DASH,UStreamTV}Segment` inherit from it and bind `Segment` to `TSegment_co`
- [ ] Add common logic for segmented stream max duration and start offset
| 2023-08-18T12:10:04 |
|
streamlink/streamlink | 5,529 | streamlink__streamlink-5529 | [
"5515"
] | 79b8497add48a7068ece0a0b2bf538db2a7d61aa | diff --git a/src/streamlink/plugins/nicolive.py b/src/streamlink/plugins/nicolive.py
--- a/src/streamlink/plugins/nicolive.py
+++ b/src/streamlink/plugins/nicolive.py
@@ -2,6 +2,9 @@
$description Japanese live-streaming and video hosting social platform.
$url live.nicovideo.jp
$type live, vod
+$metadata id
+$metadata author
+$metadata title
$account Required by some streams
$notes Timeshift is supported
"""
@@ -11,7 +14,7 @@
from threading import Event
from urllib.parse import urljoin
-from streamlink.plugin import Plugin, PluginError, pluginargument, pluginmatcher
+from streamlink.plugin import Plugin, pluginargument, pluginmatcher
from streamlink.plugin.api import useragents, validate
from streamlink.plugin.api.websocket import WebsocketClient
from streamlink.stream.hls import HLSStream, HLSStreamReader
@@ -181,7 +184,9 @@ def _get_streams(self):
self.niconico_web_login()
- wss_api_url = self.get_wss_api_url()
+ data = self.get_data()
+
+ wss_api_url = self.find_wss_api_url(data)
if not wss_api_url:
log.error(
"Failed to get wss_api_url. "
@@ -189,6 +194,8 @@ def _get_streams(self):
)
return
+ self.id, self.author, self.title = self.find_metadata(data)
+
self.wsclient = NicoLiveWsClient(self.session, wss_api_url)
self.wsclient.start()
@@ -213,26 +220,56 @@ def _get_hls_stream_url(self):
return self.wsclient.hls_stream_url
- def get_wss_api_url(self):
- try:
- data = self.session.http.get(self.url, schema=validate.Schema(
- validate.parse_html(),
- validate.xml_find(".//script[@id='embedded-data'][@data-props]"),
- validate.get("data-props"),
- validate.parse_json(),
- {"site": {
+ def get_data(self):
+ return self.session.http.get(self.url, schema=validate.Schema(
+ validate.parse_html(),
+ validate.xml_find(".//script[@id='embedded-data'][@data-props]"),
+ validate.get("data-props"),
+ validate.parse_json(),
+ ))
+
+ @staticmethod
+ def find_metadata(data):
+ schema = validate.Schema(
+ {
+ "program": {
+ "nicoliveProgramId": str,
+ "supplier": {"name": str},
+ "title": str,
+ },
+ },
+ validate.get("program"),
+ validate.union_get(
+ "nicoliveProgramId",
+ ("supplier", "name"),
+ "title",
+ ),
+ )
+
+ return schema.validate(data)
+
+ @staticmethod
+ def find_wss_api_url(data):
+ schema = validate.Schema(
+ {
+ "site": {
"relive": {
- "webSocketUrl": validate.url(scheme="wss"),
+ "webSocketUrl": validate.any(
+ validate.url(scheme="wss"),
+ "",
+ ),
},
validate.optional("frontendId"): int,
- }},
- validate.get("site"),
- validate.union_get(("relive", "webSocketUrl"), "frontendId"),
- ))
- except PluginError:
+ },
+ },
+ validate.get("site"),
+ validate.union_get(("relive", "webSocketUrl"), "frontendId"),
+ )
+
+ wss_api_url, frontend_id = schema.validate(data)
+ if not wss_api_url:
return
- wss_api_url, frontend_id = data
if frontend_id is not None:
wss_api_url = update_qsd(wss_api_url, {"frontend_id": frontend_id})
| plugins.nicolive: plugin does not support stream metadata
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
latest
### Description
Current behaviour: Author folder is not created nor is the title grabbed properly. All streams have titles on the site so not sure whats going wrong here.
Current output: -20230825200547.ts (right in the recordings folder, no author folder, no title)
Expected behaviour/output: Create Author folder and filename consisting of title-timestuff.ts
### Debug log
```text
streamlink --output "~/recordings/{author}/{title}-{time:%Y%m%d%H%M%S}.ts" https://live.nicovideo.jp/watch/co1992508 best
```
| https://streamlink.github.io/cli/metadata.html
> The availability of each variable depends on the used plugin and whether that plugin supports this kind of metadata. If a variable is unsupported or not available, then its substitution will either be a short placeholder text ([--title](https://streamlink.github.io/cli.html#cmdoption-title)) or an empty string ([--output](https://streamlink.github.io/cli.html#cmdoption-output), [--record](https://streamlink.github.io/cli.html#cmdoption-record), [--record-and-pipe](https://streamlink.github.io/cli.html#cmdoption-record-and-pipe)).
The plugin does not support any metadata:
https://github.com/streamlink/streamlink/blob/6.1.0/src/streamlink/plugins/nicolive.py#L173-L205 | 2023-09-03T14:59:48 |
|
streamlink/streamlink | 5,532 | streamlink__streamlink-5532 | [
"5531"
] | 79b8497add48a7068ece0a0b2bf538db2a7d61aa | diff --git a/src/streamlink/logger.py b/src/streamlink/logger.py
--- a/src/streamlink/logger.py
+++ b/src/streamlink/logger.py
@@ -193,7 +193,7 @@ def basicConfig(
with _config_lock:
handler: logging.StreamHandler
if filename is not None:
- handler = logging.FileHandler(filename, filemode)
+ handler = logging.FileHandler(filename, filemode, encoding="utf-8")
else:
handler = logging.StreamHandler(stream)
| diff --git a/tests/test_logger.py b/tests/test_logger.py
--- a/tests/test_logger.py
+++ b/tests/test_logger.py
@@ -5,7 +5,6 @@
from io import StringIO
from pathlib import Path
from typing import Iterable, Tuple, Type
-from unittest.mock import patch
import freezegun
import pytest
@@ -20,29 +19,53 @@ def output():
@pytest.fixture()
-def log(request, output: StringIO):
+def logfile(tmp_path: Path) -> str:
+ return str(tmp_path / "log.txt")
+
+
[email protected]()
+def log(request: pytest.FixtureRequest, monkeypatch: pytest.MonkeyPatch, output: StringIO):
params = getattr(request, "param", {})
params.setdefault("format", "[{name}][{levelname}] {message}")
params.setdefault("style", "{")
+
+ if "logfile" in request.fixturenames:
+ params["filename"] = request.getfixturevalue("logfile")
+
fakeroot = logging.getLogger("streamlink.test")
- with patch("streamlink.logger.root", fakeroot), \
- patch("streamlink.utils.times.LOCAL", timezone.utc):
- handler = logger.basicConfig(stream=output, **params)
- assert isinstance(handler, logging.Handler)
- yield fakeroot
- logger.capturewarnings(False)
- fakeroot.removeHandler(handler)
- assert not fakeroot.handlers
+
+ monkeypatch.setattr("streamlink.logger.root", fakeroot)
+ monkeypatch.setattr("streamlink.utils.times.LOCAL", timezone.utc)
+
+ handler = logger.basicConfig(stream=output, **params)
+ assert isinstance(handler, logging.Handler)
+
+ yield fakeroot
+
+ logger.capturewarnings(False)
+
+ handler.close()
+ fakeroot.removeHandler(handler)
+ assert not fakeroot.handlers
class TestLogging:
@pytest.fixture()
- def log_failure(self, request, log: logging.Logger, output: StringIO):
+ def log_failure(
+ self,
+ request: pytest.FixtureRequest,
+ monkeypatch: pytest.MonkeyPatch,
+ log: logging.Logger,
+ output: StringIO,
+ ):
params = getattr(request, "param", {})
+
root = logging.getLogger("streamlink")
- with patch("streamlink.logger.root", root):
- with pytest.raises(Exception) as cm: # noqa: PT011
- logger.basicConfig(stream=output, **params)
+ monkeypatch.setattr("streamlink.logger.root", root)
+
+ with pytest.raises(Exception) as cm: # noqa: PT011
+ logger.basicConfig(stream=output, **params)
+
return cm.value
@pytest.mark.parametrize(("name", "level"), [
@@ -164,6 +187,13 @@ def test_datefmt_custom(self, log: logging.Logger, output: StringIO):
log.info("test")
assert output.getvalue() == "[03:04:05.123456+0000][test][info] test\n"
+ def test_logfile(self, logfile: str, log: logging.Logger, output: StringIO):
+ log.setLevel("info")
+ log.info("Hello world, ΞΡιά ΟΞΏΟ
ΞΟΟΞΌΞ΅, γγγ«γ‘γ―δΈη")
+ log.handlers[0].flush()
+ with open(logfile, "r", encoding="utf-8") as fh:
+ assert fh.read() == "[test][info] Hello world, ΞΡιά ΟΞΏΟ
ΞΟΟΞΌΞ΅, γγγ«γ‘γ―δΈη\n"
+
class TestCaptureWarnings:
@staticmethod
| UnicodeEncodeError: 'charmap' codec can't encode characters
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
latest
### Description
Setting the loglevel to "info", "debug", "trace" or "all" results in an error.
See logfile
[unicode error maybe.txt](https://github.com/streamlink/streamlink/files/12506748/unicode.error.maybe.txt)
Loglevel "warning" or lower works fine.
Maybe `encoding="utf-8"` fixes this?
### Debug log
```text
streamlink -o "~/recordings/{author}/{title}-{time:%Y-%m-%d-%H-%M-%S}.ts" --logfile FILE.txt --loglevel all https://live.nicovideo.jp/watch/lv342661855 best
```
```
--- Logging error ---
Traceback (most recent call last):
File "logging\__init__.py", line 1113, in emit
File "encodings\cp1252.py", line 19, in encode
UnicodeEncodeError: 'charmap' codec can't encode characters in position 79-84: character maps to <undefined>
Call stack:
File "<frozen runpy>", line 198, in _run_module_as_main
File "<frozen runpy>", line 88, in _run_code
File "C:\Users\SecondaryH\AppData\Local\Programs\Streamlink\bin\streamlink.exe\__main__.py", line 18, in <module>
sys.exit(main())
File "C:\Users\SecondaryH\AppData\Local\Programs\Streamlink\pkgs\streamlink_cli\main.py", line 924, in main
handle_url()
File "C:\Users\SecondaryH\AppData\Local\Programs\Streamlink\pkgs\streamlink_cli\main.py", line 564, in handle_url
handle_stream(plugin, streams, stream_name)
File "C:\Users\SecondaryH\AppData\Local\Programs\Streamlink\pkgs\streamlink_cli\main.py", line 436, in handle_stream
success = output_stream(stream, formatter)
File "C:\Users\SecondaryH\AppData\Local\Programs\Streamlink\pkgs\streamlink_cli\main.py", line 332, in output_stream
output = create_output(formatter)
File "C:\Users\SecondaryH\AppData\Local\Programs\Streamlink\pkgs\streamlink_cli\main.py", line 103, in create_output
return check_file_output(formatter.path(args.output, args.fs_safe_rules), args.force)
File "C:\Users\SecondaryH\AppData\Local\Programs\Streamlink\pkgs\streamlink_cli\main.py", line 69, in check_file_output
log.info(f"Writing output to\n{path.resolve()}")
File "logging\__init__.py", line 1489, in info
File "logging\__init__.py", line 1634, in _log
File "logging\__init__.py", line 1644, in handle
File "logging\__init__.py", line 1706, in callHandlers
File "logging\__init__.py", line 978, in handle
File "logging\__init__.py", line 1230, in emit
File "logging\__init__.py", line 1118, in emit
Message: 'Writing output to\nC:\\Users\\SecondaryH\\recordings\\ε€§γ‘γγγγ³(ε€§ι士)\\22ζ³η‘θ·γ΄γη΅Άζ-2023-09-03-19-24-20.ts'
Arguments: ()
--- Logging error ---
Traceback (most recent call last):
File "logging\__init__.py", line 1113, in emit
File "encodings\cp1252.py", line 19, in encode
UnicodeEncodeError: 'charmap' codec can't encode characters in position 84-87: character maps to <undefined>
Call stack:
File "threading.py", line 995, in _bootstrap
File "threading.py", line 1038, in _bootstrap_inner
File "C:\Users\SecondaryH\AppData\Local\Programs\Streamlink\pkgs\streamlink\plugin\api\websocket.py", line 112, in run
self.ws.run_forever(**self._ws_rundata)
File "C:\Users\SecondaryH\AppData\Local\Programs\Streamlink\pkgs\websocket\_app.py", line 501, in run_forever
setSock()
File "C:\Users\SecondaryH\AppData\Local\Programs\Streamlink\pkgs\websocket\_app.py", line 428, in setSock
dispatcher.read(self.sock.sock, read, check)
File "C:\Users\SecondaryH\AppData\Local\Programs\Streamlink\pkgs\websocket\_app.py", line 99, in read
if not read_callback():
File "C:\Users\SecondaryH\AppData\Local\Programs\Streamlink\pkgs\websocket\_app.py", line 461, in read
self._callback(self.on_message, data)
File "C:\Users\SecondaryH\AppData\Local\Programs\Streamlink\pkgs\websocket\_app.py", line 547, in _callback
callback(self, *args)
File "C:\Users\SecondaryH\AppData\Local\Programs\Streamlink\pkgs\streamlink\plugins\nicolive.py", line 47, in on_message
log.debug(f"Received: {data}")
File "logging\__init__.py", line 1477, in debug
File "logging\__init__.py", line 1634, in _log
File "logging\__init__.py", line 1644, in handle
File "logging\__init__.py", line 1706, in callHandlers
File "logging\__init__.py", line 978, in handle
File "logging\__init__.py", line 1230, in emit
File "logging\__init__.py", line 1118, in emit
Message: 'Received: {"type":"room","data":{"name":"γ’γͺγΌγ","messageServer":{"uri":"wss://msgd.live2.nicovideo.jp/websocket","type":"niwavided"},"threadId":"SENSITIVE INFO","yourPostKey":"DELETED CUZ SENSiTIVE INFO I THINK","isFirst":true,"waybackkey":"waybackkey","vposBaseTime":"2023-09-03T17:42:23+09:00"}}'
Arguments: ()
```
| Not a plugin issue.
The error is triggered by the logging module and it's caused by your system's default encoding.
This got me confused for a moment, because it's actually only the logfile that's causing issues here. The regular log stream-output should be working fine.
[`logging.FileHandler()`](https://docs.python.org/3/library/logging.handlers.html#logging.FileHandler) needs the `encoding="utf-8"` arg:
https://github.com/streamlink/streamlink/blob/79b8497add48a7068ece0a0b2bf538db2a7d61aa/src/streamlink/logger.py#L194-L198 | 2023-09-03T20:19:06 |
streamlink/streamlink | 5,534 | streamlink__streamlink-5534 | [
"5524"
] | ad6952c116566ad601ada9481a9c563499c2f107 | diff --git a/src/streamlink/plugins/hiplayer.py b/src/streamlink/plugins/hiplayer.py
--- a/src/streamlink/plugins/hiplayer.py
+++ b/src/streamlink/plugins/hiplayer.py
@@ -2,7 +2,6 @@
$description United Arab Emirates CDN hosting live content for various websites in The Middle East.
$url alwasat.ly
$url media.gov.kw
-$url rotana.net
$type live
$region various
"""
@@ -19,16 +18,8 @@
log = logging.getLogger(__name__)
-@pluginmatcher(re.compile(r"""
- https?://(?:www\.)?
- (
- alwasat\.ly
- |
- media\.gov\.kw
- |
- rotana\.net
- )
-""", re.VERBOSE))
+@pluginmatcher(name="alwasatly", pattern=re.compile(r"https?://(?:www\.)?alwasat\.ly"))
+@pluginmatcher(name="mediagovkw", pattern=re.compile(r"https?://(?:www\.)?media\.gov\.kw"))
class HiPlayer(Plugin):
DAI_URL = "https://pubads.g.doubleclick.net/ssai/event/{0}/streams"
@@ -56,7 +47,7 @@ def _get_streams(self):
data = self.session.http.get(
js_url,
schema=validate.Schema(
- re.compile(r"var \w+\s*=\s*\[(?P<data>.+)]\.join\([\"']{2}\)"),
+ re.compile(r"\[(?P<data>[^]]+)]\.join\([\"']{2}\)"),
validate.none_or_all(
validate.get("data"),
validate.transform(lambda s: re.sub(r"['\", ]", "", s)),
| diff --git a/tests/plugins/test_hiplayer.py b/tests/plugins/test_hiplayer.py
--- a/tests/plugins/test_hiplayer.py
+++ b/tests/plugins/test_hiplayer.py
@@ -6,10 +6,7 @@ class TestPluginCanHandleUrlHiPlayer(PluginCanHandleUrl):
__plugin__ = HiPlayer
should_match = [
- "https://www.alwasat.ly/any",
- "https://www.alwasat.ly/any/path",
- "https://www.media.gov.kw/any",
- "https://www.media.gov.kw/any/path",
- "https://rotana.net/any",
- "https://rotana.net/any/path",
+ ("alwasatly", "https://alwasat.ly/live"),
+ ("mediagovkw", "https://www.media.gov.kw/LiveTV.aspx?PanChannel=KTV1"),
+ ("mediagovkw", "https://www.media.gov.kw/LiveTV.aspx?PanChannel=KTVSports"),
]
| plugins.hiplayer: Could not find base64 encoded JSON data
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
streamlink 6.1.0
### Description
Hi team. I was able to get stream url from following page successfully for past weeks. Two days ago, I found streamlink isn't able to retrieve the steam url:
web page: https://www.media.gov.kw/LiveTV.aspx?PanChannel=KTV1
This is the official website of Kuwait for their national channels.
I tried 3 different machines on different operating systems where streamlink was working successfully and retrieving the stream url for this website. All recently failed with same message (No playable streams...etc.)
### Debug log
```text
Desktop:~$ streamlink https://www.media.gov.kw/LiveTV.aspx?PanChannel=KTV1 --loglevel=debug
[cli][debug] OS: Linux-5.15.90.1-microsoft-standard-WSL2-x86_64-with-glibc2.35
[cli][debug] Python: 3.10.12
[cli][debug] Streamlink: 6.1.0
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.7.22
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.3
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.18.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.22.2
[cli][debug] trio-websocket: 0.10.3
[cli][debug] typing-extensions: 4.7.1
[cli][debug] urllib3: 2.0.4
[cli][debug] websocket-client: 1.6.2
[cli][debug] Arguments:
[cli][debug] url=https://www.media.gov.kw/LiveTV.aspx?PanChannel=KTV1
[cli][debug] --loglevel=debug
[cli][info] Found matching plugin hiplayer for URL https://www.media.gov.kw/LiveTV.aspx?PanChannel=KTV1
[plugins.hiplayer][debug] JS URL=https://hiplayer.hibridcdn.net/l/kwmedia-kwtv1
[plugins.hiplayer][error] Could not find base64 encoded JSON data
error: No playable streams found on this URL: https://www.media.gov.kw/LiveTV.aspx?PanChannel=KTV1
```
| 2023-09-03T23:24:37 |
|
streamlink/streamlink | 5,535 | streamlink__streamlink-5535 | [
"5530"
] | e60d7c87e200e8b60083137dc5d23d5da8a8b6e3 | diff --git a/src/streamlink_cli/argparser.py b/src/streamlink_cli/argparser.py
--- a/src/streamlink_cli/argparser.py
+++ b/src/streamlink_cli/argparser.py
@@ -427,6 +427,18 @@ def build_parser():
Default is "".
""",
)
+ player.add_argument(
+ "--player-env",
+ metavar="KEY=VALUE",
+ type=keyvalue,
+ action="append",
+ help="""
+ Add an additional environment variable to the spawned player process, in addition to the ones inherited from
+ the Streamlink/Python parent process. This allows setting player environment variables in config files.
+
+ Can be repeated to add multiple environment variables.
+ """,
+ )
player.add_argument(
"-v", "--verbose-player",
action="store_true",
diff --git a/src/streamlink_cli/main.py b/src/streamlink_cli/main.py
--- a/src/streamlink_cli/main.py
+++ b/src/streamlink_cli/main.py
@@ -140,8 +140,9 @@ def create_output(formatter: Formatter) -> Union[FileOutput, PlayerOutput]:
log.info(f"Starting player: {args.player}")
return PlayerOutput(
- args.player,
- args.player_args,
+ path=args.player,
+ args=args.player_args,
+ env=args.player_env,
quiet=not args.verbose_player,
kill=not args.player_no_close,
namedpipe=namedpipe,
@@ -187,8 +188,9 @@ def output_stream_http(
server = create_http_server()
player = output = PlayerOutput(
- args.player,
- args.player_args,
+ path=args.player,
+ args=args.player_args,
+ env=args.player_env,
quiet=not args.verbose_player,
filename=server.url,
title=formatter.title(args.title, defaults=DEFAULT_STREAM_METADATA) if args.title else args.url,
@@ -276,8 +278,9 @@ def output_stream_passthrough(stream, formatter: Formatter):
return False
output = PlayerOutput(
- args.player,
- args.player_args,
+ path=args.player,
+ args=args.player_args,
+ env=args.player_env,
quiet=not args.verbose_player,
call=True,
filename=url,
diff --git a/src/streamlink_cli/output/player.py b/src/streamlink_cli/output/player.py
--- a/src/streamlink_cli/output/player.py
+++ b/src/streamlink_cli/output/player.py
@@ -1,4 +1,5 @@
import logging
+import os
import re
import shlex
import subprocess
@@ -8,7 +9,7 @@
from pathlib import Path
from shutil import which
from time import sleep
-from typing import ClassVar, Dict, List, Optional, TextIO, Type, Union
+from typing import ClassVar, Dict, List, Mapping, Optional, Sequence, TextIO, Tuple, Type, Union
from streamlink.compat import is_win32
from streamlink.exceptions import StreamlinkWarning
@@ -158,6 +159,7 @@ def __init__(
self,
path: Path,
args: str = "",
+ env: Optional[Sequence[Tuple[str, str]]] = None,
quiet: bool = True,
kill: bool = True,
call: bool = False,
@@ -171,6 +173,8 @@ def __init__(
self.path = path
self.args = args
+ self.env: Mapping[str, str] = dict(env or {})
+
self.kill = kill
self.call = call
self.quiet = quiet
@@ -248,22 +252,30 @@ def _open(self):
self._open_subprocess(args)
def _open_call(self, args: List[str]):
- log.debug(f"Calling: {args!r}")
+ log.debug(f"Calling: {args!r}{f', env: {self.env!r}' if self.env else ''}")
+
+ environ = dict(os.environ)
+ environ.update(self.env)
subprocess.call(
args,
+ env=environ,
stdout=self.stdout,
stderr=self.stderr,
)
def _open_subprocess(self, args: List[str]):
- log.debug(f"Opening subprocess: {args!r}")
+ log.debug(f"Opening subprocess: {args!r}{f', env: {self.env!r}' if self.env else ''}")
+
+ environ = dict(os.environ)
+ environ.update(self.env)
# Force bufsize=0 on all Python versions to avoid writing the
# unflushed buffer when closing a broken input pipe
self.player = subprocess.Popen(
args,
bufsize=0,
+ env=environ,
stdin=self.stdin,
stdout=self.stdout,
stderr=self.stderr,
| diff --git a/tests/cli/output/test_player.py b/tests/cli/output/test_player.py
--- a/tests/cli/output/test_player.py
+++ b/tests/cli/output/test_player.py
@@ -1,7 +1,7 @@
import subprocess
from contextlib import nullcontext
from pathlib import Path
-from typing import ContextManager, Type
+from typing import ContextManager, Dict, Mapping, Sequence, Type
from unittest.mock import Mock, call, patch
import pytest
@@ -251,6 +251,12 @@ def test_title(self):
# TODO: refactor PlayerOutput and write proper tests
class TestPlayerOutput:
+ @pytest.fixture(autouse=True)
+ def _os_environ(self, os_environ: Dict[str, str]):
+ os_environ["FAKE"] = "ENVIRONMENT"
+ yield
+ assert sorted(os_environ.keys()) == ["FAKE"], "Doesn't pollute the os.environ dict with custom env vars"
+
@pytest.fixture()
def playeroutput(self, request: pytest.FixtureRequest):
with patch("streamlink_cli.output.player.sleep"):
@@ -269,10 +275,22 @@ def mock_popen(self, playeroutput: PlayerOutput):
patch("subprocess.Popen", return_value=Mock(poll=Mock(side_effect=Mock(return_value=None)))) as mock_popen:
yield mock_popen
- @pytest.mark.parametrize(("playeroutput", "mock_which"), [
- (
- dict(path=Path("player"), args="param1 param2", call=False),
+ @pytest.mark.parametrize(("playeroutput", "mock_which", "args", "env", "logmessage"), [
+ pytest.param(
+ {"path": Path("player"), "args": "param1 param2", "call": False},
+ "/resolved/player",
+ ["/resolved/player", "param1", "param2", "-"],
+ {"FAKE": "ENVIRONMENT"},
+ "Opening subprocess: ['/resolved/player', 'param1', 'param2', '-']",
+ id="Without custom env vars",
+ ),
+ pytest.param(
+ {"path": Path("player"), "args": "param1 param2", "env": [("VAR1", "abc"), ("VAR2", "def")], "call": False},
"/resolved/player",
+ ["/resolved/player", "param1", "param2", "-"],
+ {"FAKE": "ENVIRONMENT", "VAR1": "abc", "VAR2": "def"},
+ "Opening subprocess: ['/resolved/player', 'param1', 'param2', '-'], env: {'VAR1': 'abc', 'VAR2': 'def'}",
+ id="With custom env vars",
),
], indirect=["playeroutput", "mock_which"])
def test_open_popen_parameters(
@@ -281,6 +299,9 @@ def test_open_popen_parameters(
playeroutput: PlayerOutput,
mock_which: Mock,
mock_popen: Mock,
+ args: Sequence[str],
+ env: Mapping[str, str],
+ logmessage: str,
):
caplog.set_level(1, "streamlink")
@@ -288,12 +309,13 @@ def test_open_popen_parameters(
playeroutput.open()
assert [(record.name, record.levelname, record.message) for record in caplog.records] == [
- ("streamlink.cli.output", "debug", "Opening subprocess: ['/resolved/player', 'param1', 'param2', '-']"),
+ ("streamlink.cli.output", "debug", logmessage),
]
assert mock_which.call_args_list == [call("player")]
assert mock_popen.call_args_list == [call(
- ["/resolved/player", "param1", "param2", "-"],
+ args,
bufsize=0,
+ env=env,
stdin=subprocess.PIPE,
stdout=subprocess.DEVNULL,
stderr=subprocess.DEVNULL,
diff --git a/tests/cli/test_argparser.py b/tests/cli/test_argparser.py
--- a/tests/cli/test_argparser.py
+++ b/tests/cli/test_argparser.py
@@ -1,5 +1,4 @@
-import argparse
-from argparse import ArgumentParser, Namespace
+from argparse import Namespace
from pathlib import Path
from typing import Any, List
from unittest.mock import Mock
@@ -7,7 +6,7 @@
import pytest
from streamlink.session import Streamlink
-from streamlink_cli.argparser import build_parser, setup_session_options
+from streamlink_cli.argparser import ArgumentParser, build_parser, setup_session_options
from streamlink_cli.main import main as streamlink_cli_main
@@ -18,7 +17,7 @@ def parser():
class TestConfigFileArguments:
@pytest.fixture()
- def parsed(self, request: pytest.FixtureRequest, parser: argparse.ArgumentParser, tmp_path: Path):
+ def parsed(self, request: pytest.FixtureRequest, parser: ArgumentParser, tmp_path: Path):
content = "\n".join([
"",
" ",
@@ -74,6 +73,13 @@ def test_withvalue(self, parsed: Namespace):
def test_emptyvalue(self, parsed: Namespace):
assert parsed.title == ""
+ @pytest.mark.parametrize("parsed", [
+ pytest.param(["http-header=foo=bar=baz", "http-header=FOO=BAR=BAZ"], id="With operator"),
+ pytest.param(["http-header foo=bar=baz", "http-header FOO=BAR=BAZ"], id="Without operator"),
+ ], indirect=True)
+ def test_keyequalsvalue(self, parsed: Namespace):
+ assert parsed.http_header == [("foo", "bar=baz"), ("FOO", "BAR=BAZ")]
+
@pytest.mark.filterwarnings("ignore")
@pytest.mark.parametrize(("argv", "option", "expected"), [
diff --git a/tests/cli/test_cmdline.py b/tests/cli/test_cmdline.py
--- a/tests/cli/test_cmdline.py
+++ b/tests/cli/test_cmdline.py
@@ -46,9 +46,9 @@ def fn(*_):
assert exit_code == actual_exit_code
assert mock_setup_streamlink.call_count == 1
if not passthrough:
- assert mock_popen.call_args_list == [call(commandline, bufsize=ANY, stdin=ANY, stdout=ANY, stderr=ANY)]
+ assert mock_popen.call_args_list == [call(commandline, env=ANY, bufsize=ANY, stdin=ANY, stdout=ANY, stderr=ANY)]
else:
- assert mock_call.call_args_list == [call(commandline, stdout=ANY, stderr=ANY)]
+ assert mock_call.call_args_list == [call(commandline, env=ANY, stdout=ANY, stderr=ANY)]
class TestCommandLine(CommandLineTestCase):
diff --git a/tests/cli/test_main.py b/tests/cli/test_main.py
--- a/tests/cli/test_main.py
+++ b/tests/cli/test_main.py
@@ -289,10 +289,12 @@ def test_create_output_no_file_output_options(self, args: Mock):
args.url = "URL"
args.player = Path("mpv")
args.player_args = ""
+ args.player_env = None
output = create_output(formatter)
assert type(output) is PlayerOutput
assert output.playerargs.title == "URL"
+ assert output.env == {}
args.title = "{author} - {title}"
output = create_output(formatter)
@@ -379,12 +381,14 @@ def test_create_output_record(self, mock_check_file_output: Mock, args: Mock):
args.url = "URL"
args.player = Path("mpv")
args.player_args = ""
+ args.player_env = [("VAR1", "abc"), ("VAR2", "def")]
args.player_fifo = None
args.player_http = None
output = create_output(formatter)
assert type(output) is PlayerOutput
assert output.playerargs.title == "URL"
+ assert output.env == {"VAR1": "abc", "VAR2": "def"}
assert type(output.record) is FileOutput
assert output.record.filename == Path("foo")
assert output.record.fd is None
@@ -415,12 +419,14 @@ def test_create_output_record_stdout(self, args: Mock):
args.url = "URL"
args.player = Path("mpv")
args.player_args = ""
+ args.player_env = [("VAR1", "abc"), ("VAR2", "def")]
args.player_fifo = None
args.player_http = None
output = create_output(formatter)
assert type(output) is PlayerOutput
assert output.playerargs.title == "foo - bar"
+ assert output.env == {"VAR1": "abc", "VAR2": "def"}
assert type(output.record) is FileOutput
assert output.record.filename is None
assert output.record.fd is stdout
diff --git a/tests/conftest.py b/tests/conftest.py
--- a/tests/conftest.py
+++ b/tests/conftest.py
@@ -83,3 +83,17 @@ def requests_mock(requests_mock: rm.Mocker) -> rm.Mocker:
"""
requests_mock.register_uri(rm.ANY, rm.ANY, exc=rm.exceptions.InvalidRequest)
return requests_mock
+
+
[email protected]()
+def os_environ(request: pytest.FixtureRequest, monkeypatch: pytest.MonkeyPatch) -> Dict[str, str]:
+ class FakeEnviron(dict):
+ def __setitem__(self, key, value):
+ if key == "PYTEST_CURRENT_TEST":
+ return
+ return super().__setitem__(key, value)
+
+ fakeenviron = FakeEnviron(getattr(request, "param", {}))
+ monkeypatch.setattr("os.environ", fakeenviron)
+
+ return fakeenviron
| Allow setting environment variables when launching the player
### Checklist
- [X] This is a feature request and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed feature requests](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22feature+request%22)
### Description
There is currently the `--player-arg` that allows to pass argument to the player.
It would be very handy to have another one, `--player-env` to enable setting environment variables when the player is launched.
I currently have only a single use case:
- I use pipewire, and I want to hint pipewire to connect a specific node, this is done via the `PIPEWIRE_NODE` environment variable.
I can set the environment variable before launching streamlink, but this requires editing all my launchers, instead of setting it in the config file.
| Child processes inherit the environment of the parent process unless it gets explicitly overridden, which Streamlink does not:
https://github.com/streamlink/streamlink/blob/79b8497add48a7068ece0a0b2bf538db2a7d61aa/src/streamlink_cli/output/player.py#L264-L270
Just set the env var(s) on the streamlink/python process.
@bastimeyer Yes I know as I said at the bottom of my initial issue, but the idea was to be able to use streamlink own config file. I guess I can work around by wrapping my launchers.
Ah, sorry, apparently I didn't finish reading the whole post :/
A new CLI arg could be added, similar to how `--http-header` works (multiple CLI args with `key=value` values that get appended), but I fear that we'd very likely see requests for adding such args for every other kind of child process Streamlink spawns, not just the player.
IMO, adding specific env vars just for the player is kind of an edge case, so writing a player wrapper script or streamlink wrapper script is probably the better idea.
I can see having a specific CLI arg being useful from the perspective of a config file though.
Yes the main point here is to be able to use a config file to pass environment variable to the player. It is an edge case, and I do not have many use cases for now. The only one I can think of is to configure libraries used by players, I mention pipewire which is an actual use case. But there are other things that can only be configured with env var:
- selecting wayland or xorg, with things like GDK_BACKEND or QT_QPA_PLATFORM
- QT or GTK theming
- locale
- SDL based players as SDL use many env vars
now the main point is: does it make sense to change those for streamlink only. In my case yes, as I want mpv to use a different audio sink when launched from streamlink but not when used alone.
I also opened the issue for reference and in case that someone else has the same problem. I realize this might be out of scope and it is perfectly fine if you close it.
I guess the best workaround would be to write a script, like `mpv_st` which sets env var before opening the player. It does require a bit of care to ensure args and stdin is handled correctly. | 2023-09-04T22:34:13 |
streamlink/streamlink | 5,538 | streamlink__streamlink-5538 | [
"5536"
] | c6cd719369fa611b964545c22bca8f0c00d153d4 | diff --git a/src/streamlink/stream/hls.py b/src/streamlink/stream/hls.py
--- a/src/streamlink/stream/hls.py
+++ b/src/streamlink/stream/hls.py
@@ -477,13 +477,15 @@ def iter_segments(self):
log.info(f"Stopping stream early after {self.duration_limit}")
return
- # End of stream
- stream_end = self.playlist_end is not None and sequence.num >= self.playlist_end
- if self.closed or stream_end:
+ if self.closed: # pragma: no cover
return
self.playlist_sequence = sequence.num + 1
+ # End of stream
+ if self.closed or self.playlist_end is not None and (not queued or self.playlist_sequence > self.playlist_end):
+ return
+
if queued:
self.playlist_sequences_last = now()
elif self._segment_queue_timing_threshold_reached():
| diff --git a/tests/stream/test_hls.py b/tests/stream/test_hls.py
--- a/tests/stream/test_hls.py
+++ b/tests/stream/test_hls.py
@@ -142,7 +142,14 @@ def get_session(self, options=None, *args, **kwargs):
def test_playlist_end(self):
thread, segments = self.subject([
- # media sequence = 0
+ Playlist(0, [Segment(0)], end=True),
+ ])
+
+ assert self.await_read(read_all=True) == self.content(segments), "Stream ends and read-all handshake doesn't time out"
+
+ def test_playlist_end_on_empty_reload(self):
+ thread, segments = self.subject([
+ Playlist(0, [Segment(0)]),
Playlist(0, [Segment(0)], end=True),
])
@@ -377,6 +384,7 @@ def test_playlist_reload_offset(self) -> None:
# time_completed = 00:00:01; time_elapsed = 1s
assert worker.handshake_wait.wait_ready(1), "Arrives at first wait() call"
assert worker.playlist_sequence == 1, "Has queued first segment"
+ assert worker.playlist_end is None, "Stream hasn't ended yet"
assert worker.time_wait == 4.0, "Waits for 4 seconds out of the 5 seconds reload time"
self.await_playlist_wait()
@@ -392,6 +400,7 @@ def test_playlist_reload_offset(self) -> None:
# time_completed = 00:00:12; time_elapsed = 7s (exceeded 5s targetduration)
assert worker.handshake_wait.wait_ready(1), "Arrives at second wait() call"
assert worker.playlist_sequence == 2, "Has queued second segment"
+ assert worker.playlist_end is None, "Stream hasn't ended yet"
assert worker.time_wait == 0.0, "Doesn't wait when reloading took too long"
self.await_playlist_wait()
@@ -407,6 +416,7 @@ def test_playlist_reload_offset(self) -> None:
# time_completed = 00:00:13; time_elapsed = 1s
assert worker.handshake_wait.wait_ready(1), "Arrives at third wait() call"
assert worker.playlist_sequence == 3, "Has queued third segment"
+ assert worker.playlist_end is None, "Stream hasn't ended yet"
assert worker.time_wait == 4.0, "Waits for 4 seconds out of the 5 seconds reload time"
self.await_playlist_wait()
@@ -422,6 +432,7 @@ def test_playlist_reload_offset(self) -> None:
# time_completed = 00:00:17; time_elapsed = 0s
assert worker.handshake_wait.wait_ready(1), "Arrives at fourth wait() call"
assert worker.playlist_sequence == 4, "Has queued fourth segment"
+ assert worker.playlist_end is None, "Stream hasn't ended yet"
assert worker.time_wait == 5.0, "Waits for the whole reload time"
self.await_playlist_wait()
@@ -433,7 +444,7 @@ def test_playlist_reload_offset(self) -> None:
self.await_playlist_reload()
assert self.await_read(read_all=True) == self.content(segments)
self.await_close()
- assert worker.playlist_sequence == 4, "Doesn't update sequence number once ended"
+ assert worker.playlist_end == 4, "Stream has ended"
assert not worker.handshake_wait.wait_ready(0), "Doesn't wait once ended"
assert not worker.handshake_reload.wait_ready(0), "Doesn't reload playlist once ended"
| HLS streams sometimes fail to end after encountering #EXT-X-ENDLIST
### Checklist
- [X] This is a bug report and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed bug reports](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22bug%22)
- [x] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
streamlink 6.1.0+20.ge60d7c87
### Description
Occasionally, HLS streams will keep trying to refresh the playlist even after encountering `#EXT-X-ENDLIST`. This will continue until some other mechanism kicks in to kill the stream (read timeout, queue threshold, etc). This doesn't happen every single time, only occasionally.
I was logging with loglevel=all so the log is very verbose, I've cut out parts I believe are redundant, also cut out sensitive information.
I've found similar issues at #3628 and #2198, but both of them claim it was caused by `#EXT-X-ENDLIST` being missing from the playlist, so they're different from this case.
### Debug log
```text
[05:02:27.624459][cli][debug] OS: Linux-6.1.44-x86_64-with-glibc2.24
[05:02:27.624924][cli][debug] Python: 3.11.0
[05:02:27.625185][cli][debug] OpenSSL: OpenSSL 1.1.1s 1 Nov 2022
[05:02:27.625459][cli][debug] Streamlink: 6.1.0+20.ge60d7c87
[05:02:27.626924][cli][debug] Dependencies:
[05:02:27.658497][cli][debug] certifi: 2022.9.24
[05:02:27.660236][cli][debug] isodate: 0.6.1
[05:02:27.667291][cli][debug] lxml: 4.9.1
[05:02:27.677015][cli][debug] pycountry: 22.3.5
[05:02:27.685567][cli][debug] pycryptodome: 3.15.0
[05:02:27.688960][cli][debug] PySocks: 1.7.1
[05:02:27.690272][cli][debug] requests: 2.28.1
[05:02:27.700613][cli][debug] trio: 0.22.2
[05:02:27.706916][cli][debug] trio-websocket: 0.10.3
[05:02:27.721277][cli][debug] typing-extensions: 4.7.1
[05:02:27.740413][cli][debug] urllib3: 1.26.12
[05:02:27.750012][cli][debug] websocket-client: 1.4.2
[05:02:27.750634][cli][debug] Arguments:
[05:02:27.750929][cli][debug] url=https://www.twitch.tv/user
[05:02:27.751172][cli][debug] stream=['best']
[05:02:27.751485][cli][debug] --loglevel=all
[05:02:27.751779][cli][debug] --logfile=user/2023_09_06_05_02_26_(UTC)_29227.ts.log
[05:02:27.752114][cli][debug] --output=user/2023_09_06_05_02_26_(UTC)_29227.ts
[05:02:27.752373][cli][debug] --retry-streams=5.0
[05:02:27.752609][cli][debug] --retry-max=12
[05:02:27.752936][cli][debug] --retry-open=10
[05:02:27.753178][cli][debug] --stream-segment-attempts=20
[05:02:27.753382][cli][debug] --stream-segment-threads=1
[05:02:27.753616][cli][debug] --stream-segment-timeout=60.0
[05:02:27.753897][cli][debug] --stream-timeout=240.0
[05:02:27.754093][cli][debug] --hls-playlist-reload-attempts=10
[05:02:27.754236][cli][debug] --hls-playlist-reload-time=segment
[05:02:27.754471][cli][debug] --hls-segment-queue-threshold=0.0
[05:02:27.754754][cli][debug] --hls-live-restart=True
[05:02:27.754937][cli][debug] --webbrowser=False
[05:02:27.755176][cli][debug] --twitch-api-header=[('Authorization', 'OAuth '), ('Client-ID', '')]
[05:02:27.755329][cli][debug] --twitch-access-token-param=[('playerType', ''), ('platform', '')]
[05:02:27.756035][cli][info] Found matching plugin twitch for URL https://www.twitch.tv/user
[05:02:27.756229][plugins.twitch][debug] Getting live HLS streams for user
[05:02:28.013601][plugins.twitch][debug] {'adblock': False, 'geoblock_reason': '', 'hide_ads': False, 'server_ads': True, 'show_ads': True}
[05:02:28.194074][utils.l10n][debug] Language code: en_GB
...
[05:02:28.661391][cli][info] Available streams: audio_only, 160p (worst), 360p, 480p, 720p60, 1080p60 (best)
[05:02:28.661602][cli][info] Opening stream: 1080p60 (hls)
[05:02:28.662044][cli][info] Writing output to
...
[05:02:28.662216][cli][debug] Checking file output
[05:02:28.663634][stream.hls][debug] Reloading playlist
[05:02:28.663938][cli][debug] Pre-buffering 8192 bytes
[05:02:29.804419][stream.hls_playlist][all] #EXT-X-VERSION:3
[05:02:29.804803][stream.hls_playlist][all] #EXT-X-TARGETDURATION:6
[05:02:29.805119][stream.hls_playlist][all] #EXT-X-MEDIA-SEQUENCE:0
[05:02:29.805369][stream.hls_playlist][all] #EXT-X-TWITCH-LIVE-SEQUENCE:0
[05:02:29.805602][stream.hls_playlist][all] #EXT-X-TWITCH-ELAPSED-SECS:0.000
[05:02:29.805853][stream.hls_playlist][all] #EXT-X-TWITCH-TOTAL-SECS:10.000
[05:02:29.806088][stream.hls_playlist][all] #EXT-X-DATERANGE:ID="playlist-creation-1693976549",CLASS="timestamp",START-DATE="2023-09-05T22:02:29.739-07:00",END-ON-NEXT=YES,X-SERVER-TIME="1693976549.74"
[05:02:29.823833][stream.hls_playlist][all] #EXT-X-DATERANGE:ID="playlist-session-1693976549",CLASS="twitch-session",START-DATE="2023-09-05T22:02:29.739-07:00",END-ON-NEXT=YES,X-TV-TWITCH-SESSIONID="..."
[05:02:29.824331][stream.hls_playlist][all] #EXT-X-DATERANGE:ID="source-1693976542",CLASS="twitch-stream-source",START-DATE="2023-09-06T05:02:22.709Z",END-ON-NEXT=YES,X-TV-TWITCH-STREAM-SOURCE="live"
[05:02:29.824671][stream.hls_playlist][all] #EXT-X-DATERANGE:ID="trigger-1693976542",CLASS="twitch-trigger",START-DATE="2023-09-06T05:02:22.709Z",END-ON-NEXT=YES,X-TV-TWITCH-TRIGGER-URL="https://video-weaver....Iwuwc"
[05:02:29.824988][stream.hls_playlist][all] #EXT-X-PROGRAM-DATE-TIME:2023-09-06T05:02:22.709Z
[05:02:29.825203][stream.hls_playlist][all] #EXTINF:2.000,live
[05:02:29.825424][stream.hls_playlist][all] https://video-edge-...dXMtd2VzdC0yMLsH.ts
[05:02:29.825682][stream.hls_playlist][all] #EXT-X-PROGRAM-DATE-TIME:2023-09-06T05:02:24.709Z
[05:02:29.825955][stream.hls_playlist][all] #EXTINF:2.000,live
[05:02:29.826242][stream.hls_playlist][all] https://video-edge-...Kgl1cy13ZXN0LTIwuwc.ts
[05:02:29.826528][stream.hls_playlist][all] #EXT-X-PROGRAM-DATE-TIME:2023-09-06T05:02:26.709Z
[05:02:29.826785][stream.hls_playlist][all] #EXTINF:2.000,live
[05:02:29.827001][stream.hls_playlist][all] https://video-edge-...y13ZXN0LTIwuwc.ts
[05:02:29.827297][stream.hls_playlist][all] #EXT-X-TWITCH-PREFETCH:https://video-edge-...1cy13ZXN0LTIwuwc.ts
[05:02:29.827529][stream.hls_playlist][all] #EXT-X-TWITCH-PREFETCH:https://video-edge-...1cy13ZXN0LTIwuwc.ts
[05:02:29.827938][stream.hls][debug] First Sequence: 0; Last Sequence: 2
[05:02:29.828114][stream.hls][debug] Start offset: 0; Duration: None; Start Sequence: 0; End Sequence: None
[05:02:29.828282][stream.hls][debug] Adding segment 0 to queue
[05:02:29.829283][stream.hls][debug] Adding segment 1 to queue
[05:02:29.831928][stream.hls][debug] Adding segment 2 to queue
[05:02:30.663827][stream.hls][debug] Reloading playlist
... (stream continues normally) ...
[06:03:02.663152][stream.hls][debug] Reloading playlist
[06:03:03.067024][stream.hls_playlist][all] #EXT-X-VERSION:3
[06:03:03.067433][stream.hls_playlist][all] #EXT-X-TARGETDURATION:6
[06:03:03.067698][stream.hls_playlist][all] #EXT-X-MEDIA-SEQUENCE:1802
[06:03:03.067965][stream.hls_playlist][all] #EXT-X-TWITCH-LIVE-SEQUENCE:1802
[06:03:03.068209][stream.hls_playlist][all] #EXT-X-TWITCH-ELAPSED-SECS:3604.000
[06:03:03.068424][stream.hls_playlist][all] #EXT-X-TWITCH-TOTAL-SECS:3634.990
[06:03:03.068650][stream.hls_playlist][all] #EXT-X-DATERANGE:ID="playlist-creation-1693976549",CLASS="timestamp",START-DATE="2023-09-06T05:02:29.739Z",END-ON-NEXT=YES,X-SERVER-TIME="1693976549.74"
[06:03:03.069041][stream.hls_playlist][all] #EXT-X-DATERANGE:ID="playlist-session-1693976549",CLASS="twitch-session",START-DATE="2023-09-06T05:02:29.739Z",END-ON-NEXT=YES,X-TV-TWITCH-SESSIONID="..."
[06:03:03.069400][stream.hls_playlist][all] #EXT-X-DATERANGE:ID="source-1693976542",CLASS="twitch-stream-source",START-DATE="2023-09-06T05:02:22.709Z",END-ON-NEXT=YES,X-TV-TWITCH-STREAM-SOURCE="live"
[06:03:03.069770][stream.hls_playlist][all] #EXT-X-DATERANGE:ID="trigger-1693976542",CLASS="twitch-trigger",START-DATE="2023-09-06T05:02:22.709Z",END-ON-NEXT=YES,X-TV-TWITCH-TRIGGER-URL="https://video-weaver....Iwuwc"
[06:03:03.070091][stream.hls_playlist][all] #EXT-X-PROGRAM-DATE-TIME:2023-09-06T06:02:26.709Z
[06:03:03.070390][stream.hls_playlist][all] #EXTINF:2.000,live
[06:03:03.070680][stream.hls_playlist][all] https://video-edge-....ts
[06:03:03.070980][stream.hls_playlist][all] #EXT-X-PROGRAM-DATE-TIME:2023-09-06T06:02:28.709Z
[06:03:03.071231][stream.hls_playlist][all] #EXTINF:2.000,live
[06:03:03.071493][stream.hls_playlist][all] https://video-edge-....ts
[06:03:03.071730][stream.hls_playlist][all] #EXT-X-PROGRAM-DATE-TIME:2023-09-06T06:02:30.709Z
[06:03:03.071994][stream.hls_playlist][all] #EXTINF:2.000,live
[06:03:03.072272][stream.hls_playlist][all] https://video-edge-....ts
[06:03:03.072511][stream.hls_playlist][all] #EXT-X-PROGRAM-DATE-TIME:2023-09-06T06:02:32.709Z
[06:03:03.072863][stream.hls_playlist][all] #EXTINF:2.000,live
[06:03:03.073797][stream.hls_playlist][all] https://video-edge-....ts
[06:03:03.074266][stream.hls_playlist][all] #EXT-X-PROGRAM-DATE-TIME:2023-09-06T06:02:34.709Z
[06:03:03.074597][stream.hls_playlist][all] #EXTINF:2.000,live
[06:03:03.074968][stream.hls_playlist][all] https://video-edge-....ts
[06:03:03.075538][stream.hls_playlist][all] #EXT-X-PROGRAM-DATE-TIME:2023-09-06T06:02:36.709Z
[06:03:03.075771][stream.hls_playlist][all] #EXTINF:2.000,live
[06:03:03.076017][stream.hls_playlist][all] https://video-edge-....ts
[06:03:03.076302][stream.hls_playlist][all] #EXT-X-PROGRAM-DATE-TIME:2023-09-06T06:02:38.709Z
[06:03:03.076847][stream.hls_playlist][all] #EXTINF:2.000,live
[06:03:03.077096][stream.hls_playlist][all] https://video-edge-....ts
[06:03:03.077312][stream.hls_playlist][all] #EXT-X-PROGRAM-DATE-TIME:2023-09-06T06:02:40.709Z
[06:03:03.077547][stream.hls_playlist][all] #EXTINF:2.000,live
[06:03:03.077828][stream.hls_playlist][all] https://video-edge-....ts
[06:03:03.078035][stream.hls_playlist][all] #EXT-X-PROGRAM-DATE-TIME:2023-09-06T06:02:42.709Z
[06:03:03.078249][stream.hls_playlist][all] #EXTINF:2.000,live
[06:03:03.078518][stream.hls_playlist][all] https://video-edge-....ts
[06:03:03.078821][stream.hls_playlist][all] #EXT-X-PROGRAM-DATE-TIME:2023-09-06T06:02:44.709Z
[06:03:03.079106][stream.hls_playlist][all] #EXTINF:2.000,live
[06:03:03.079383][stream.hls_playlist][all] https://video-edge-....ts
[06:03:03.079643][stream.hls_playlist][all] #EXT-X-PROGRAM-DATE-TIME:2023-09-06T06:02:46.709Z
[06:03:03.079952][stream.hls_playlist][all] #EXTINF:2.000,live
[06:03:03.080207][stream.hls_playlist][all] https://video-edge-....ts
[06:03:03.080468][stream.hls_playlist][all] #EXT-X-PROGRAM-DATE-TIME:2023-09-06T06:02:48.709Z
[06:03:03.080775][stream.hls_playlist][all] #EXTINF:2.000,live
[06:03:03.080994][stream.hls_playlist][all] https://video-edge-....ts
[06:03:03.081184][stream.hls_playlist][all] #EXT-X-PROGRAM-DATE-TIME:2023-09-06T06:02:50.709Z
[06:03:03.081369][stream.hls_playlist][all] #EXTINF:2.000,live
[06:03:03.081562][stream.hls_playlist][all] https://video-edge-....ts
[06:03:03.081793][stream.hls_playlist][all] #EXT-X-PROGRAM-DATE-TIME:2023-09-06T06:02:52.709Z
[06:03:03.082044][stream.hls_playlist][all] #EXTINF:2.000,live
[06:03:03.082322][stream.hls_playlist][all] https://video-edge-....ts
[06:03:03.082507][stream.hls_playlist][all] #EXT-X-PROGRAM-DATE-TIME:2023-09-06T06:02:54.709Z
[06:03:03.082786][stream.hls_playlist][all] #EXTINF:1.990,live
[06:03:03.083076][stream.hls_playlist][all] https://video-edge-....ts
[06:03:03.083357][stream.hls_playlist][all] #EXT-X-PROGRAM-DATE-TIME:2023-09-06T06:02:56.699Z
[06:03:03.083652][stream.hls_playlist][all] #EXTINF:1.000,live
[06:03:03.083942][stream.hls_playlist][all] https://video-edge-....ts
[06:03:03.084164][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:03.663126][stream.hls][debug] Reloading playlist
...
[06:03:03.810475][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:04.663097][stream.hls][debug] Reloading playlist
...
[06:03:05.158972][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:05.663178][stream.hls][debug] Reloading playlist
...
[06:03:05.814325][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:06.663167][stream.hls][debug] Reloading playlist
...
[06:03:07.145406][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:07.663106][stream.hls][debug] Reloading playlist
...
[06:03:07.806248][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:08.663129][stream.hls][debug] Reloading playlist
...
[06:03:08.806112][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:09.663137][stream.hls][debug] Reloading playlist
...
[06:03:09.847093][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:10.663126][stream.hls][debug] Reloading playlist
...
[06:03:10.807157][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:11.663171][stream.hls][debug] Reloading playlist
...
[06:03:11.835474][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:12.663121][stream.hls][debug] Reloading playlist
...
[06:03:12.805984][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:13.663117][stream.hls][debug] Reloading playlist
...
[06:03:13.845596][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:14.663132][stream.hls][debug] Reloading playlist
...
[06:03:14.806936][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:15.663139][stream.hls][debug] Reloading playlist
...
[06:03:15.837861][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:16.663162][stream.hls][debug] Reloading playlist
...
[06:03:16.807616][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:17.663268][stream.hls][debug] Reloading playlist
...
[06:03:17.855211][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:18.663123][stream.hls][debug] Reloading playlist
...
[06:03:18.809816][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:19.663106][stream.hls][debug] Reloading playlist
...
[06:03:19.841545][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:20.663269][stream.hls][debug] Reloading playlist
...
[06:03:20.807515][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:21.663114][stream.hls][debug] Reloading playlist
...
[06:03:21.845081][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:22.663117][stream.hls][debug] Reloading playlist
...
[06:03:22.808568][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:23.663105][stream.hls][debug] Reloading playlist
...
[06:03:23.839342][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:24.663108][stream.hls][debug] Reloading playlist
...
[06:03:24.813164][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:25.663126][stream.hls][debug] Reloading playlist
...
[06:03:25.840110][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:26.663163][stream.hls][debug] Reloading playlist
...
[06:03:26.807925][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:27.663105][stream.hls][debug] Reloading playlist
...
[06:03:27.840355][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:28.663155][stream.hls][debug] Reloading playlist
...
[06:03:28.806406][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:29.663111][stream.hls][debug] Reloading playlist
...
[06:03:29.847565][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:30.663112][stream.hls][debug] Reloading playlist
...
[06:03:30.804173][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:31.663100][stream.hls][debug] Reloading playlist
...
[06:03:31.839352][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:32.663195][stream.hls][debug] Reloading playlist
...
[06:03:32.804562][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:33.663118][stream.hls][debug] Reloading playlist
...
[06:03:33.842320][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:34.663112][stream.hls][debug] Reloading playlist
...
[06:03:34.805074][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:35.663097][stream.hls][debug] Reloading playlist
...
[06:03:35.804672][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:36.663130][stream.hls][debug] Reloading playlist
[06:04:41.463081][stream.hls][warning] Failed to reload playlist: Unable to open URL: https://video-weaver.....m3u8 (404 Client Error: Not Found for url: https://video-weaver.....m3u8)
[06:04:41.463501][stream.hls][debug] Reloading playlist
[06:05:46.361899][stream.hls][warning] Failed to reload playlist: Unable to open URL: https://video-weaver.....m3u8 (404 Client Error: Not Found for url: https://video-weaver.....m3u8)
[06:05:46.383504][stream.hls][debug] Reloading playlist
[06:06:51.409273][stream.hls][warning] Failed to reload playlist: Unable to open URL: https://video-weaver.....m3u8 (404 Client Error: Not Found for url: https://video-weaver.....m3u8)
[06:06:51.409991][stream.hls][debug] Reloading playlist
[06:06:59.977777][stream.segmented][debug] Closing worker thread
[06:06:59.978834][stream.segmented][debug] Closing writer thread
[06:07:56.289789][stream.hls][warning] Failed to reload playlist: Unable to open URL: https://video-weaver.....m3u8 (404 Client Error: Not Found for url: https://video-weaver.....m3u8)
[06:07:56.311945][cli][info] Stream ended
error: Error when reading from stream: Read timeout, exiting
[06:07:56.316438][cli][info] Closing currently open stream...
```
| Thinking about it, the logs immediately preceding the EXT-X-ENDLIST may be useful as well, I had originally cut them thinking they were redundant but stuff like the segment numbers may be useful, so here are those as well:
```log
[06:02:51.805036][stream.hls][debug] Adding segment 1813 to queue
[06:02:52.184364][stream.hls][debug] Writing segment 1813 to output
[06:02:52.187681][stream.hls][debug] Segment 1813 complete
[06:02:53.663803][stream.hls][debug] Reloading playlist
[06:02:53.793217][stream.hls_playlist][all] #EXT-X-VERSION:3
[06:02:53.793525][stream.hls_playlist][all] #EXT-X-TARGETDURATION:6
[06:02:53.793722][stream.hls_playlist][all] #EXT-X-MEDIA-SEQUENCE:1801
[06:02:53.793936][stream.hls_playlist][all] #EXT-X-TWITCH-LIVE-SEQUENCE:1801
...
[06:02:53.805896][stream.hls][debug] Adding segment 1814 to queue
[06:02:54.033768][stream.hls][debug] Writing segment 1814 to output
[06:02:54.042736][stream.hls][debug] Segment 1814 complete
[06:02:55.663795][stream.hls][debug] Reloading playlist
[06:02:55.793338][stream.hls_playlist][all] #EXT-X-VERSION:3
[06:02:55.793686][stream.hls_playlist][all] #EXT-X-TARGETDURATION:6
[06:02:55.793952][stream.hls_playlist][all] #EXT-X-MEDIA-SEQUENCE:1802
[06:02:55.794148][stream.hls_playlist][all] #EXT-X-TWITCH-LIVE-SEQUENCE:1802
...
[06:02:55.806166][stream.hls][debug] Adding segment 1815 to queue
[06:02:56.490534][stream.hls][debug] Writing segment 1815 to output
[06:02:56.496597][stream.hls][debug] Segment 1815 complete
[06:02:57.663801][stream.hls][debug] Reloading playlist
[06:02:57.792855][stream.hls_playlist][all] #EXT-X-VERSION:3
[06:02:57.793142][stream.hls_playlist][all] #EXT-X-TARGETDURATION:6
[06:02:57.793382][stream.hls_playlist][all] #EXT-X-MEDIA-SEQUENCE:1802
[06:02:57.793541][stream.hls_playlist][all] #EXT-X-TWITCH-LIVE-SEQUENCE:1802
...
[06:02:57.804605][stream.hls][debug] Adding segment 1816 to queue
[06:02:58.009763][stream.hls][debug] Writing segment 1816 to output
[06:02:58.012956][stream.hls][debug] Segment 1816 complete
[06:02:59.663139][stream.hls][debug] Reloading playlist
[06:02:59.792351][stream.hls_playlist][all] #EXT-X-VERSION:3
[06:02:59.792676][stream.hls_playlist][all] #EXT-X-TARGETDURATION:6
[06:02:59.792904][stream.hls_playlist][all] #EXT-X-MEDIA-SEQUENCE:1802
[06:02:59.793068][stream.hls_playlist][all] #EXT-X-TWITCH-LIVE-SEQUENCE:1802
...
[06:02:59.804388][stream.hls][debug] Adding segment 1817 to queue
[06:02:59.973558][stream.hls][debug] Writing segment 1817 to output
[06:02:59.974804][stream.hls][debug] Segment 1817 complete
[06:03:00.663144][stream.hls][debug] Reloading playlist
[06:03:00.794212][stream.hls_playlist][all] #EXT-X-VERSION:3
[06:03:00.794557][stream.hls_playlist][all] #EXT-X-TARGETDURATION:6
[06:03:00.794836][stream.hls_playlist][all] #EXT-X-MEDIA-SEQUENCE:1802
[06:03:00.795031][stream.hls_playlist][all] #EXT-X-TWITCH-LIVE-SEQUENCE:1802
...
[06:03:01.668640][stream.hls][debug] Reloading playlist
[06:03:01.800664][stream.hls_playlist][all] #EXT-X-VERSION:3
[06:03:01.801168][stream.hls_playlist][all] #EXT-X-TARGETDURATION:6
[06:03:01.801425][stream.hls_playlist][all] #EXT-X-MEDIA-SEQUENCE:1802
[06:03:01.801774][stream.hls_playlist][all] #EXT-X-TWITCH-LIVE-SEQUENCE:1802
...
[06:03:02.663152][stream.hls][debug] Reloading playlist
[06:03:03.067024][stream.hls_playlist][all] #EXT-X-VERSION:3
[06:03:03.067433][stream.hls_playlist][all] #EXT-X-TARGETDURATION:6
[06:03:03.067698][stream.hls_playlist][all] #EXT-X-MEDIA-SEQUENCE:1802
[06:03:03.067965][stream.hls_playlist][all] #EXT-X-TWITCH-LIVE-SEQUENCE:1802
...
[06:03:03.084164][stream.hls_playlist][all] #EXT-X-ENDLIST
[06:03:03.663126][stream.hls][debug] Reloading playlist
```
`EXT-X-ENDLIST` sets `m3u8.is_endlist` to `True`
https://github.com/streamlink/streamlink/blob/c6cd719369fa611b964545c22bca8f0c00d153d4/src/streamlink/stream/hls_playlist.py#L484-L490
When `m3u8.is_endlist == True`, set `playlist_end` to the final sequence number
https://github.com/streamlink/streamlink/blob/c6cd719369fa611b964545c22bca8f0c00d153d4/src/streamlink/stream/hls.py#L390-L391
`playlist_end` is checked here, inside the `playlist_sequences` iterator loop.
https://github.com/streamlink/streamlink/blob/c6cd719369fa611b964545c22bca8f0c00d153d4/src/streamlink/stream/hls.py#L470-L483
So the above case seems to be an edge case where `#EXT-X-ENDLIST` is appended to the playlist without any new segments being added, so the `playlist_end` check is never done.
It seems like `playlist_end` should be checked somewhere outside of the sequence iterator loop.
> So the above case seems to be an edge case where `#EXT-X-ENDLIST` is appended to the playlist without any new segments being added, so the `playlist_end` check is never done.
Yes, that seems to be correct. Thanks for checking.
Fixing this should be pretty straightforward. I'll have a look once I get the time. I'm currently not at my computer and will be busy first with fixing a Streamlink Twitch GUI emergency issue when I come home. | 2023-09-06T21:04:47 |
streamlink/streamlink | 5,551 | streamlink__streamlink-5551 | [
"5546"
] | 9a3583d7d94f5923d3d1c136546193e39c3c2d3e | diff --git a/src/streamlink/plugins/pluto.py b/src/streamlink/plugins/pluto.py
--- a/src/streamlink/plugins/pluto.py
+++ b/src/streamlink/plugins/pluto.py
@@ -10,7 +10,7 @@
import logging
import re
-from urllib.parse import parse_qs, urljoin
+from urllib.parse import parse_qsl, urljoin
from uuid import uuid4
from streamlink.plugin import Plugin, pluginmatcher
@@ -23,7 +23,7 @@
class PlutoHLSStreamWriter(HLSStreamWriter):
- ad_re = re.compile(r"_ad/creative/|dai\.google\.com|Pluto_TV_OandO/.*Bumper")
+ ad_re = re.compile(r"_ad/creative/|dai\.google\.com|Pluto_TV_OandO/.*(Bumper|plutotv_filler)")
def should_filter_sequence(self, sequence):
return self.ad_re.search(sequence.segment.uri) is not None or super().should_filter_sequence(sequence)
@@ -115,9 +115,13 @@ def _get_api_data(self, kind, slug, slugfilter=None):
)
def _get_playlist(self, host, path, params, token):
- qs = parse_qs(params)
- qs["jwt"] = token
- yield from PlutoHLSStream.parse_variant_playlist(self.session, update_qsd(urljoin(host, path), qs)).items()
+ qsd = dict(parse_qsl(params))
+ qsd["jwt"] = token
+
+ url = urljoin(host, path)
+ url = update_qsd(url, qsd)
+
+ return PlutoHLSStream.parse_variant_playlist(self.session, url)
@staticmethod
def _get_media_data(data, key, slug):
| plugins.Pluto: Freezes at commercials and plays no audio on TV Shows
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [x] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest build from the master branch
### Description
Hello! Pluto TV plugin have problems. The plugin plays fine, but it freezes at commercials and some TV shows don't have commercials, it plays no audio because it thinks it at a commercial. This happens on all Pluto TV channels.
### Debug log
```text
C:\Windows\system32>streamlink -l debug https://pluto.tv/live-tv/forever-kids best
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.11.5
[cli][debug] Streamlink: 6.1.0
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.7.22
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.3
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.18.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.22.2
[cli][debug] trio-websocket: 0.10.3
[cli][debug] typing-extensions: 4.7.1
[cli][debug] urllib3: 2.0.4
[cli][debug] websocket-client: 1.6.1
[cli][debug] Arguments:
[cli][debug] url=https://pluto.tv/live-tv/forever-kids
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --ffmpeg-ffmpeg=C:\Program Files (x86)\Streamlink\ffmpeg\ffmpeg.exe
[cli][info] Found matching plugin pluto for URL https://pluto.tv/live-tv/forever-kids
[plugins.pluto][debug] slug=forever-kids
[plugins.pluto][debug] app_version=7.7.0-18f7ab32608969ea5bcbce8d0e23b9d0e1b24717
[stream.hls][warning] Encountered a stream discontinuity. This is unsupported and will result in incoherent output data.
```
| > it freezes at commercials
Which is intended behavior of this plugin's custom HLS implementation, as you can see here:
https://github.com/streamlink/streamlink/blame/6.1.0/src/streamlink/plugins/pluto.py#L21-L25
> it plays no audio
Try using a different player. These are unmuxed streams, so the output will be exactly the same as the site provides. Stream discontinuities cause issues with the playback. The warning message tells you that the output is incoherent.
> [stream.hls][warning] Encountered a stream discontinuity. This is unsupported and will result in incoherent output data.
This comes from the fact that segments get filtered out.
See #4535.
> some TV shows don't have commercials
I can't judge this since I don't use the site/plugin, but the filtering segment URL regex linked above doesn't look like it would do that.
Post the full log output of those streams then. The log output you've posted is incomplete, despite the issue template explicitly telling you not to remove stuff.
Setting the log level to `all` ([`--loglevel=all`](https://streamlink.github.io/cli.html#cmdoption-loglevel)) will show you the contents of each HLS playlist request, which is very verbose, but it'll let you see which segments will get filtered out.
Had another look and it seems like the plugin is incorrectly setting query string parameters on the HLS playlist request, which results in the streaming server returning different content.
This here is incorrect:
https://github.com/streamlink/streamlink/blame/6.1.0/src/streamlink/plugins/pluto.py#L114-L115
It should be `dict(parse_qsl(...))`. | 2023-09-12T23:58:02 |
|
streamlink/streamlink | 5,557 | streamlink__streamlink-5557 | [
"5556"
] | 51a9ba8d20b490b892d89412962f2ebc38d611d0 | diff --git a/src/streamlink/plugins/goodgame.py b/src/streamlink/plugins/goodgame.py
--- a/src/streamlink/plugins/goodgame.py
+++ b/src/streamlink/plugins/goodgame.py
@@ -1,71 +1,89 @@
"""
-$description Russian live streaming platform for live video game broadcasts.
+$description Russian live-streaming platform for live video game broadcasts.
$url goodgame.ru
$type live
+$metadata id
+$metadata author
+$metadata category
+$metadata title
"""
import logging
import re
+import sys
+from urllib.parse import urlparse, urlunparse
from streamlink.plugin import Plugin, pluginmatcher
+from streamlink.plugin.api import validate
from streamlink.stream.hls import HLSStream
-from streamlink.utils.parse import parse_json
log = logging.getLogger(__name__)
-HLS_URL_FORMAT = "https://hls.goodgame.ru/hls/{0}{1}.m3u8"
-QUALITIES = {
- "1080p": "",
- "720p": "_720",
- "480p": "_480",
- "240p": "_240",
-}
-
-_apidata_re = re.compile(r"""(?P<quote>["']?)channel(?P=quote)\s*:\s*(?P<data>{.*?})\s*,""")
-_ddos_re = re.compile(r'document.cookie="(__DDOS_[^;]+)')
-
@pluginmatcher(re.compile(
- r"https?://(?:www\.)?goodgame\.ru/channel/(?P<user>[^/]+)",
+ r"https?://(?:www\.)?goodgame\.ru/(?:channel/)?(?P<user>[^/]+)",
))
class GoodGame(Plugin):
- def _check_stream(self, url):
- res = self.session.http.get(url, acceptable_status=(200, 404))
- if res.status_code == 200:
- return True
+ @classmethod
+ def stream_weight(cls, stream):
+ if stream == "source":
+ return sys.maxsize, stream
+ return super().stream_weight(stream)
- def _get_streams(self):
- headers = {
- "Referer": self.url,
- }
- res = self.session.http.get(self.url, headers=headers)
+ def __init__(self, *args, **kwargs):
+ super().__init__(*args, **kwargs)
- match = _ddos_re.search(res.text)
- if match:
- log.debug("Anti-DDOS bypass...")
- headers["Cookie"] = match.group(1)
- res = self.session.http.get(self.url, headers=headers)
+ # `/channel/user` returns different JSON data with less useful information
+ url = urlparse(self.url)
+ self.url = urlunparse(url._replace(path=re.sub(r"^/channel/", "/", url.path)))
- match = _apidata_re.search(res.text)
- channel_info = match and parse_json(match.group("data"))
- if not channel_info:
+ def _get_streams(self):
+ data = self.session.http.get(
+ self.url,
+ schema=validate.Schema(
+ validate.parse_html(),
+ validate.xml_xpath_string(".//script[contains(text(),'channel:{')][1]/text()"),
+ re.compile(r"channel:(?P<json>{.+?}),?\n"),
+ validate.none_or_all(
+ validate.get("json"),
+ validate.parse_json(),
+ {
+ "status": bool,
+ "id": int,
+ "streamer": {"username": str},
+ "game": str,
+ "title": str,
+ "sources": validate.all(
+ {str: validate.url()},
+ validate.filter(lambda k, v: ".m3u8" in v),
+ ),
+ },
+ validate.union_get(
+ "status",
+ "id",
+ ("streamer", "username"),
+ "game",
+ "title",
+ "sources",
+ ),
+ ),
+ ),
+ )
+
+ if not data:
log.error("Could not find channel info")
return
- log.debug("Found channel info: id={id} channelkey={channelkey} pid={streamkey} online={status}".format(**channel_info))
- if not channel_info["status"]:
- log.debug("Channel appears to be offline")
-
- streams = {}
- for name, url_suffix in QUALITIES.items():
- url = HLS_URL_FORMAT.format(channel_info["streamkey"], url_suffix)
- if not self._check_stream(url):
- continue
+ status, self.id, self.author, self.category, self.title, sources = data
- streams[name] = HLSStream(self.session, url)
+ if not status:
+ log.debug("Channel appears to be offline")
+ return
- return streams
+ for name, url in sources.items():
+ name = f"{name}{'p' if name.isnumeric() else ''}"
+ yield name, HLSStream(self.session, url)
__plugin__ = GoodGame
| diff --git a/tests/plugins/test_goodgame.py b/tests/plugins/test_goodgame.py
--- a/tests/plugins/test_goodgame.py
+++ b/tests/plugins/test_goodgame.py
@@ -1,13 +1,23 @@
+import pytest
+
from streamlink.plugins.goodgame import GoodGame
+from streamlink.session import Streamlink
from tests.plugins import PluginCanHandleUrl
class TestPluginCanHandleUrlGoodGame(PluginCanHandleUrl):
__plugin__ = GoodGame
- should_match = [
- "https://goodgame.ru/channel/ABC_ABC/#autoplay",
- "https://goodgame.ru/channel/ABC123ABC/#autoplay",
- "https://goodgame.ru/channel/ABC/#autoplay",
- "https://goodgame.ru/channel/123ABC123/#autoplay",
+ should_match_groups = [
+ ("https://goodgame.ru/CHANNELNAME", {"user": "CHANNELNAME"}),
+ ("https://goodgame.ru/channel/CHANNELNAME", {"user": "CHANNELNAME"}),
]
+
+
[email protected](("url", "expected"), [
+ ("https://goodgame.ru/CHANNELNAME", "https://goodgame.ru/CHANNELNAME"),
+ ("https://goodgame.ru/channel/CHANNELNAME", "https://goodgame.ru/CHANNELNAME"),
+])
+def test_url_translation(session: Streamlink, url: str, expected: str):
+ plugin = GoodGame(session, url)
+ assert plugin.url == expected
| plugins.goodgame: Unable to parse JSON: Expecting ','
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Streamlink: 6.2.0
### Description
Hello,
if put full url of goodgame stream (old version of url on site) (https://goodgame.ru/channel/melharucos), then goes error "Unable to parse JSON: Expecting ',' delimiter: line 1 column 689 (char 688) ('{"id":171713,"title":"melharucos", ...)".
if put shorten url (new version of url on site) (https://goodgame.ru/melharucos), then goes error "error: No plugin can handle URL: https://goodgame.ru/melharucos".
### Debug log
```text
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.11.5
[cli][debug] OpenSSL: OpenSSL 3.0.9 30 May 2023
[cli][debug] Streamlink: 6.2.0
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.7.22
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.3
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.18.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.22.2
[cli][debug] trio-websocket: 0.10.4
[cli][debug] typing-extensions: 4.7.1
[cli][debug] urllib3: 2.0.4
[cli][debug] websocket-client: 1.6.3
[cli][debug] Arguments:
[cli][debug] url=https://goodgame.ru/channel/melharucos
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --ffmpeg-ffmpeg=C:\Soft\Streamlink\ffmpeg\ffmpeg.exe
[cli][info] Found matching plugin goodgame for URL https://goodgame.ru/channel/melharucos
error: Unable to parse JSON: Expecting ',' delimiter: line 1 column 689 (char 688) ('{"id":171713,"title":"melharucos", ...)
or
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.11.5
[cli][debug] OpenSSL: OpenSSL 3.0.9 30 May 2023
[cli][debug] Streamlink: 6.2.0
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.7.22
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.3
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.18.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.22.2
[cli][debug] trio-websocket: 0.10.4
[cli][debug] typing-extensions: 4.7.1
[cli][debug] urllib3: 2.0.4
[cli][debug] websocket-client: 1.6.3
[cli][debug] Arguments:
[cli][debug] url=https://goodgame.ru/melharucos
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --ffmpeg-ffmpeg=C:\Soft\Streamlink\ffmpeg\ffmpeg.exe
error: No plugin can handle URL: https://goodgame.ru/melharucos
```
| The plugin hasn't been updated in a long time and needs a rewrite. The site seems to be very simple though, so a rewrite should be fairly trivial. I'll have a look later. | 2023-09-15T20:49:08 |
streamlink/streamlink | 5,559 | streamlink__streamlink-5559 | [
"5503"
] | 97b5e181a545a9e18b21b18c53632865735b61a2 | diff --git a/src/streamlink/plugins/wwenetwork.py b/src/streamlink/plugins/wwenetwork.py
--- a/src/streamlink/plugins/wwenetwork.py
+++ b/src/streamlink/plugins/wwenetwork.py
@@ -8,7 +8,6 @@
import json
import logging
import re
-from functools import lru_cache
from urllib.parse import parse_qsl, urlparse
from streamlink.plugin import Plugin, PluginError, pluginargument, pluginmatcher
@@ -21,7 +20,7 @@
@pluginmatcher(re.compile(
- r"https?://watch\.wwe\.com/(channel)?",
+ r"https?://network\.wwe\.com/(?:video|live)/(?P<stream_id>\d+)",
))
@pluginargument(
"email",
@@ -42,7 +41,7 @@ class WWENetwork(Plugin):
stream_url = "https://dce-frontoffice.imggaming.com/api/v2/stream/{id}"
live_url = "https://dce-frontoffice.imggaming.com/api/v2/event/live"
login_url = "https://dce-frontoffice.imggaming.com/api/v2/login"
- page_config_url = "https://cdn.watch.wwe.com/api/page"
+
API_KEY = "cca51ea0-7837-40df-a055-75eb6347b2e7"
customer_id = 16
@@ -52,14 +51,11 @@ def __init__(self, *args, **kwargs):
self.session.http.headers.update({"User-Agent": useragents.CHROME})
self.auth_token = None
- def get_title(self):
- return self.item_config["title"]
-
def request(self, method, url, **kwargs):
headers = kwargs.pop("headers", {})
headers.update({"x-api-key": self.API_KEY,
- "Origin": "https://watch.wwe.com",
- "Referer": "https://watch.wwe.com/signin",
+ "Origin": "https://network.wwe.com",
+ "Referer": "https://network.wwe.com/signin",
"Accept": "application/json",
"Realm": "dce.wwe"})
if self.auth_token:
@@ -89,25 +85,6 @@ def login(self, email, password):
return self.auth_token
- @property # type: ignore
- @lru_cache(maxsize=128) # noqa: B019
- def item_config(self):
- log.debug("Loading page config")
- p = urlparse(self.url)
- res = self.session.http.get(self.page_config_url,
- params=dict(device="web_browser",
- ff="idp,ldp",
- item_detail_expand="all",
- lang="en-US",
- list_page_size="1",
- max_list_prefetch="1",
- path=p.path,
- segments="es",
- sub="Registered",
- text_entry_format="html"))
- data = self.session.http.json(res)
- return data["item"]
-
def _get_media_info(self, content_id):
"""
Get the info about the content, based on the ID
@@ -117,23 +94,19 @@ def _get_media_info(self, content_id):
info = self.request("GET", self.stream_url.format(id=content_id))
return self.request("GET", info.get("playerUrlCallback"))
- def _get_video_id(self):
- # check the page to find the contentId
- log.debug("Searching for content ID")
- try:
- if self.item_config["type"] == "channel":
- return self._get_live_id()
- else:
- return "vod/{id}".format(id=self.item_config["customFields"]["DiceVideoId"])
- except KeyError:
- log.error("Could not find video ID")
- return
+ def _get_video_id(self, stream_id):
+ live_id = self._get_live_id(stream_id)
+ if not live_id:
+ return "vod/{0}".format(stream_id)
+
+ return live_id
- def _get_live_id(self):
+ def _get_live_id(self, stream_id):
log.debug("Loading live event")
res = self.request("GET", self.live_url)
for event in res.get("events", []):
- return "event/{sportId}/{propertyId}/{tournamentId}/{id}".format(**event)
+ if str(event["id"]) == stream_id:
+ return "event/{sportId}/{propertyId}/{tournamentId}/{id}".format(**event)
def _get_streams(self):
if not self.login(self.get_option("email"), self.get_option("password")):
@@ -146,7 +119,8 @@ def _get_streams(self):
except ValueError:
start_point = 0
- content_id = self._get_video_id()
+ stream_id = self.match.group("stream_id")
+ content_id = self._get_video_id(stream_id)
if content_id:
log.debug("Found content ID: {0}".format(content_id))
| diff --git a/tests/plugins/test_wwenetwork.py b/tests/plugins/test_wwenetwork.py
--- a/tests/plugins/test_wwenetwork.py
+++ b/tests/plugins/test_wwenetwork.py
@@ -5,6 +5,9 @@
class TestPluginCanHandleUrlWWENetwork(PluginCanHandleUrl):
__plugin__ = WWENetwork
- should_match = [
- "https://watch.wwe.com/in-ring/3622",
+
+ should_match_groups = [
+ ("https://network.wwe.com/video/3622", {"stream_id": "3622"}),
+ ("https://network.wwe.com/live/3622", {"stream_id": "3622"}),
]
+
| plugins.wwenetwork: No plugin can handle URL
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
streamlink 6.1.0
### Description
When attempting to open a WWE Network stream, I get an error 'No plugin can handle URL' occurs.
I'm not sure, but it looks like the WWE Network has changed the layout of their urls from https://watch.wwe.com/channel/2026 to https://network.wwe.com/video/505406/
### Debug log
```text
C:\Website\Streams>echo off
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.11.4
[cli][debug] Streamlink: 6.1.0
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.7.22
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.3
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.18.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.22.2
[cli][debug] trio-websocket: 0.10.3
[cli][debug] typing-extensions: 4.7.1
[cli][debug] urllib3: 2.0.4
[cli][debug] websocket-client: 1.6.1
[cli][debug] Arguments:
[cli][debug] url=https://network.wwe.com/video/70713/st-valentines-day-massacre-1999
[cli][debug] stream=['720p']
[cli][debug] --loglevel=debug
[cli][debug] --output=C:\Website\WWE-2023-08-17-17-11-36.mp4
[cli][debug] --ffmpeg-ffmpeg=C:\Program Files\Streamlink\ffmpeg\ffmpeg.exe
[cli][debug] --wwenetwork-email=*******
[cli][debug] --wwenetwork-password=********
error: No plugin can handle URL: https://network.wwe.com/video/70713/st-valentines-day-massacre-1999
Press any key to continue
```
| 2023-09-17T19:15:58 |
|
streamlink/streamlink | 5,565 | streamlink__streamlink-5565 | [
"5555"
] | 22f191705ef6ae1ab61081034a2921fa3d2de976 | diff --git a/src/streamlink/plugins/nos.py b/src/streamlink/plugins/nos.py
--- a/src/streamlink/plugins/nos.py
+++ b/src/streamlink/plugins/nos.py
@@ -2,6 +2,7 @@
$description Live TV channels and video on-demand service from NOS, a Dutch public, state-owned broadcaster.
$url nos.nl
$type live, vod
+$metadata id
$metadata title
$region Netherlands
"""
@@ -9,7 +10,7 @@
import logging
import re
-from streamlink.plugin import Plugin, PluginError, pluginmatcher
+from streamlink.plugin import Plugin, pluginmatcher
from streamlink.plugin.api import validate
from streamlink.stream.hls import HLSStream
@@ -18,86 +19,43 @@
@pluginmatcher(re.compile(
- r"https?://(?:\w+\.)?nos\.nl/(?:livestream|collectie|video|uitzendingen)",
+ r"https?://(?:\w+\.)?nos\.nl/(?:live|video|collectie)",
))
class NOS(Plugin):
- _msg_live_offline = "This livestream is offline."
- vod_keys = {
- "pages/Collection/Video/Video": "item",
- "pages/Video/Video": "video",
- }
-
def _get_streams(self):
- try:
- scripts = self.session.http.get(self.url, schema=validate.Schema(
+ data = self.session.http.get(
+ self.url,
+ schema=validate.Schema(
validate.parse_html(),
- validate.xml_findall(".//script[@type='application/json'][@data-ssr-name]"),
- [
- validate.union((
- validate.get("data-ssr-name"),
- validate.all(
- validate.getattr("text"),
- validate.parse_json(),
- ),
- )),
- ],
- ))
- except PluginError:
- log.error("Could not find any stream data")
- return
-
- for _data_ssr_name, _data_json in scripts:
- video_url = None
- log.trace(f"Found _data_ssr_name={_data_ssr_name}")
-
- if _data_ssr_name == "pages/Broadcasts/Broadcasts":
- self.title, video_url, is_live = validate.Schema(
- {"currentLivestream": {
- "is_live": bool,
- "title": str,
- "stream": validate.url(),
- }},
- validate.get("currentLivestream"),
- validate.union_get("title", "stream", "is_live"),
- ).validate(_data_json)
- if not is_live:
- log.error(self._msg_live_offline)
- continue
-
- elif _data_ssr_name == "pages/Livestream/Livestream":
- self.title, video_url, is_live = validate.Schema(
+ validate.xml_xpath_string(".//script[@type='application/ld+json'][1]/text()"),
+ validate.none_or_all(
+ validate.parse_json(),
{
- "streamIsLive": bool,
- "title": str,
- "stream": validate.url(),
+ "@type": "VideoObject",
+ "encodingFormat": "application/vnd.apple.mpegurl",
+ "contentUrl": validate.url(),
+ "identifier": validate.any(int, str),
+ "name": str,
},
- validate.union_get("title", "stream", "streamIsLive"),
- ).validate(_data_json)
- if not is_live:
- log.error(self._msg_live_offline)
- continue
+ validate.union_get(
+ "contentUrl",
+ "identifier",
+ "name",
+ ),
+ ),
+ ),
+ )
+ if not data:
+ return
- elif _data_ssr_name in self.vod_keys.keys():
- _key = self.vod_keys[_data_ssr_name]
- self.title, video_url = validate.Schema(
- {_key: {
- "title": str,
- "aspect_ratios": {
- "profiles": validate.all(
- [{
- "name": str,
- "url": validate.url(),
- }],
- validate.filter(lambda p: p["name"] == "hls_unencrypted"),
- ),
- },
- }},
- validate.get(_key),
- validate.union_get("title", ("aspect_ratios", "profiles", 0, "url")),
- ).validate(_data_json)
+ hls_url, self.id, self.title = data
+
+ res = self.session.http.get(hls_url, raise_for_status=False)
+ if res.status_code >= 400:
+ log.error("Content is inaccessible or may have expired")
+ return
- if video_url is not None:
- return HLSStream.parse_variant_playlist(self.session, video_url)
+ return HLSStream.parse_variant_playlist(self.session, hls_url)
__plugin__ = NOS
| diff --git a/tests/plugins/test_nos.py b/tests/plugins/test_nos.py
--- a/tests/plugins/test_nos.py
+++ b/tests/plugins/test_nos.py
@@ -6,15 +6,17 @@ class TestPluginCanHandleUrlNOS(PluginCanHandleUrl):
__plugin__ = NOS
should_match = [
- "https://nos.nl/livestream/2220100-wk-sprint-schaatsen-1-000-meter-mannen.html",
+ "https://nos.nl/live",
"https://nos.nl/collectie/13781/livestream/2385081-ek-voetbal-engeland-schotland",
- "https://nos.nl/collectie/13781/livestream/2385461-ek-voetbal-voorbeschouwing-italie-wales-18-00-uur",
- "https://nos.nl/collectie/13781/video/2385846-ek-in-2-21-gosens-show-tegen-portugal-en-weer-volle-bak-in-boedapest",
- "https://nos.nl/video/2385779-dronebeelden-tonen-spoor-van-vernieling-bij-leersum",
- "https://nos.nl/uitzendingen",
- "https://nos.nl/uitzendingen/livestream/2385462",
+ "https://nos.nl/collectie/13951/video/2491092-dit-was-prinsjesdag",
+ "https://nos.nl/video/2490788-meteoor-gespot-boven-noord-nederland",
]
should_not_match = [
"https://nos.nl/artikel/2385784-explosieve-situatie-leidde-tot-verwoeste-huizen-en-omgewaaide-bomen-leersum",
+ "https://nos.nl/sport",
+ "https://nos.nl/sport/videos",
+ "https://nos.nl/programmas",
+ "https://nos.nl/uitzendingen",
+ "https://nos.nl/uitzendingen/livestream/2385462",
]
| plugins.nos: No playable streams found on this URL
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
streamlink 6.1.0
### Description
None of the livestreams, collections or article videos on NOS.nl seem to be recognized (anymore).
Example video: https://nos.nl/l/2490404
Example collection: https://nos.nl/collectie/13950-natuurramp-libie
For current example livestreams, see https://nos.nl/live
### Debug log
```text
streamlink --loglevel=debug https://nos.nl/livestream/2489911
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.11.5
[cli][debug] Streamlink: 6.1.0
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.7.22
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.3
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.18.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.22.2
[cli][debug] trio-websocket: 0.10.3
[cli][debug] typing-extensions: 4.7.1
[cli][debug] urllib3: 2.0.4
[cli][debug] websocket-client: 1.6.1
[cli][debug] Arguments:
[cli][debug] url=https://nos.nl/livestream/2489911
[cli][debug] --config=['D:\\Scoop\\apps\\streamlink\\current\\config']
[cli][debug] --loglevel=debug
[cli][debug] --ffmpeg-ffmpeg=D:\Scoop\apps\streamlink\current\ffmpeg\ffmpeg.exe
[cli][info] Found matching plugin nos for URL https://nos.nl/livestream/2489911
error: No playable streams found on this URL: https://nos.nl/livestream/2489911
```
| 2023-09-21T13:10:27 |
|
streamlink/streamlink | 5,569 | streamlink__streamlink-5569 | [
"5568"
] | ff3c47d91361b62e655462e9879406377e073701 | diff --git a/src/streamlink/plugins/pandalive.py b/src/streamlink/plugins/pandalive.py
--- a/src/streamlink/plugins/pandalive.py
+++ b/src/streamlink/plugins/pandalive.py
@@ -18,7 +18,7 @@
@pluginmatcher(re.compile(
- r"https?://(?:www\.)?pandalive\.co\.kr/",
+ r"https?://(?:www\.)?pandalive\.co\.kr/live/play/[^/]+",
))
class Pandalive(Plugin):
def _get_streams(self):
@@ -34,10 +34,14 @@ def _get_streams(self):
json = self.session.http.post(
"https://api.pandalive.co.kr/v1/live/play",
+ headers={
+ "Referer": self.url,
+ },
data={
"action": "watch",
"userId": media_code,
},
+ acceptable_status=(200, 400),
schema=validate.Schema(
validate.parse_json(),
validate.any(
| diff --git a/tests/plugins/test_pandalive.py b/tests/plugins/test_pandalive.py
--- a/tests/plugins/test_pandalive.py
+++ b/tests/plugins/test_pandalive.py
@@ -6,12 +6,6 @@ class TestPluginCanHandleUrlPandalive(PluginCanHandleUrl):
__plugin__ = Pandalive
should_match = [
- "http://pandalive.co.kr/",
- "http://pandalive.co.kr/any/path",
- "http://www.pandalive.co.kr/",
- "http://www.pandalive.co.kr/any/path",
- "https://pandalive.co.kr/",
- "https://pandalive.co.kr/any/path",
- "https://www.pandalive.co.kr/",
- "https://www.pandalive.co.kr/any/path",
+ "https://www.pandalive.co.kr/live/play/pocet00",
+ "https://www.pandalive.co.kr/live/play/rladbfl1208",
]
| plugins.pandalive: HTTP status 400 on API call
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
streamlink 6.2.0
### Description
the livestreams can't resolved on pandalive
it was always resolved 400 Client Error
### Debug log
```text
C:\Users\Jerry>C:\APP\Streamlink\bin\streamlink.exe https://www.pandalive.co.kr/live/play/pocet00 --loglevel=debug
[session][debug] Plugin pandalive is being overridden by C:\Users\Jerry\AppData\Roaming\streamlink\plugins\pandalive.py
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.11.5
[cli][debug] OpenSSL: OpenSSL 3.0.9 30 May 2023
[cli][debug] Streamlink: 6.2.0
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.7.22
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.3
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.18.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.22.2
[cli][debug] trio-websocket: 0.10.4
[cli][debug] typing-extensions: 4.7.1
[cli][debug] urllib3: 2.0.4
[cli][debug] websocket-client: 1.6.3
[cli][debug] Arguments:
[cli][debug] url=https://www.pandalive.co.kr/live/play/pocet00
[cli][debug] --loglevel=debug
[cli][debug] --ffmpeg-ffmpeg=C:\APP\Streamlink\ffmpeg\ffmpeg.exe
[cli][info] Found matching plugin pandalive for URL https://www.pandalive.co.kr/live/play/pocet00
[plugins.pandalive][debug] Media code: pocet00
error: Unable to open URL: https://api.pandalive.co.kr/v1/live/play (400 Client Error: Bad Request for url: https://api.pandalive.co.kr/v1/live/play)
```
| > [session][debug] Plugin pandalive is being overridden by C:\Users\Jerry\AppData\Roaming\streamlink\plugins\pandalive.py
You are sideloading a different version of that plugin from a custom source.
https://streamlink.github.io/cli/plugin-sideloading.html
There won't be any support for custom plugin versions.
The issue however also exists on the regular plugin, which was updated last a year ago in #4591. | 2023-09-24T14:20:16 |
streamlink/streamlink | 5,586 | streamlink__streamlink-5586 | [
"5583"
] | 5f64f2ee3a4d436cd6686efb2353109fd4da4b70 | diff --git a/src/streamlink/plugins/goodgame.py b/src/streamlink/plugins/goodgame.py
--- a/src/streamlink/plugins/goodgame.py
+++ b/src/streamlink/plugins/goodgame.py
@@ -10,9 +10,9 @@
import logging
import re
-import sys
-from urllib.parse import urlparse, urlunparse
+from urllib.parse import parse_qsl, urlparse
+from streamlink.exceptions import NoStreamsError, PluginError
from streamlink.plugin import Plugin, pluginmatcher
from streamlink.plugin.api import validate
from streamlink.stream.hls import HLSStream
@@ -21,69 +21,125 @@
log = logging.getLogger(__name__)
-@pluginmatcher(re.compile(
- r"https?://(?:www\.)?goodgame\.ru/(?:channel/)?(?P<user>[^/]+)",
+@pluginmatcher(name="default", pattern=re.compile(
+ r"https?://(?:www\.)?goodgame\.ru/(?P<name>(?!channel|player)[^/?]+)",
+))
+@pluginmatcher(name="channel", pattern=re.compile(
+ r"https?://(?:www\.)?goodgame\.ru/channel/(?P<channel>[^/?]+)",
+))
+@pluginmatcher(name="player", pattern=re.compile(
+ r"https?://(?:www\.)?goodgame\.ru/player\?(?P<id>\d+)$",
))
class GoodGame(Plugin):
- @classmethod
- def stream_weight(cls, stream):
- if stream == "source":
- return sys.maxsize, stream
- return super().stream_weight(stream)
+ _API_STREAMS_ID = "https://goodgame.ru/api/4/streams/2/id/{id}"
+ _API_STREAMS_CHANNEL = "https://goodgame.ru/api/4/streams/2/channel/{channel}"
+ _URL_HLS = "https://hls.goodgame.ru/manifest/{id}_master.m3u8"
- def __init__(self, *args, **kwargs):
- super().__init__(*args, **kwargs)
+ def _get_channel_key(self):
+ return self.session.http.get(self.url, schema=validate.Schema(
+ re.compile(r"api:(?P<json>{.+?}),\n"),
+ validate.none_or_all(
+ validate.get("json"),
+ validate.parse_json(),
+ {"channel_key": str},
+ validate.get("channel_key"),
+ ),
+ ))
- # `/channel/user` returns different JSON data with less useful information
- url = urlparse(self.url)
- self.url = urlunparse(url._replace(path=re.sub(r"^/channel/", "/", url.path)))
+ def _get_api_url(self):
+ if self.matches["default"]:
+ channel = self._get_channel_key()
+ log.debug(f"{channel=}")
+ if not channel:
+ raise NoStreamsError
+ return self._API_STREAMS_CHANNEL.format(channel=channel)
- def _get_streams(self):
- data = self.session.http.get(
- self.url,
- schema=validate.Schema(
- validate.parse_html(),
- validate.xml_xpath_string(".//script[contains(text(),'channel:{')][1]/text()"),
- re.compile(r"channel:(?P<json>{.+?}),?\n"),
- validate.none_or_all(
- validate.get("json"),
- validate.parse_json(),
+ elif self.matches["channel"]:
+ return self._API_STREAMS_CHANNEL.format(channel=self.match["channel"])
+
+ elif self.matches["player"]:
+ return self._API_STREAMS_ID.format(id=self.match["id"])
+
+ raise PluginError("Invalid matcher")
+
+ def _api_stream(self, url):
+ return self.session.http.get(url, schema=validate.Schema(
+ validate.parse_json(),
+ validate.any(
+ validate.all(
{
- "status": bool,
+ "error": str,
+ },
+ validate.get("error"),
+ validate.transform(lambda data: ("error", data)),
+ ),
+ validate.all(
+ {
+ "online": bool,
"id": int,
- "streamer": {"username": str},
- "game": str,
- "title": str,
- "sources": validate.all(
- {str: validate.url()},
- validate.filter(lambda k, v: ".m3u8" in v),
- ),
+ "streamer": {
+ "username": str,
+ },
+ "game": {
+ "title": validate.none_or_all(str),
+ },
+ "title": validate.none_or_all(str),
+ "players": [
+ validate.all(
+ {
+ "title": str,
+ "online": bool,
+ "content": validate.all(
+ str,
+ validate.parse_html(),
+ validate.xml_find(".//iframe"),
+ validate.get("src"),
+ validate.transform(urlparse),
+ ),
+ },
+ validate.union_get(
+ "title",
+ "online",
+ "content",
+ ),
+ ),
+ ],
},
validate.union_get(
- "status",
+ "online",
"id",
("streamer", "username"),
- "game",
+ ("game", "title"),
"title",
- "sources",
+ "players",
),
+ validate.transform(lambda data: ("data", *data)),
),
),
- )
+ ))
- if not data:
- log.error("Could not find channel info")
+ def _get_streams(self):
+ api_url = self._get_api_url()
+ log.debug(f"{api_url=}")
+
+ result, *data = self._api_stream(api_url)
+ if result == "error":
+ log.error(data[0] or "Unknown error")
return
- status, self.id, self.author, self.category, self.title, sources = data
+ online, self.id, self.author, self.category, self.title, players = data
+ hls_url = self._URL_HLS.format(id=self.id)
- if not status:
- log.debug("Channel appears to be offline")
- return
+ if online and self.session.http.get(hls_url, raise_for_status=False).status_code < 400:
+ return HLSStream.parse_variant_playlist(self.session, hls_url)
- for name, url in sources.items():
- name = f"{name}{'p' if name.isnumeric() else ''}"
- yield name, HLSStream(self.session, url)
+ log.debug("Channel is offline, checking for embedded players...")
+ for p_title, p_online, p_url in players:
+ if p_title == "Twitch" and p_online:
+ channel = dict(parse_qsl(p_url.query)).get("channel")
+ if channel:
+ log.debug(f"Redirecting to Twitch: {channel=}")
+ return self.session.streams(f"twitch.tv/{channel}")
__plugin__ = GoodGame
| diff --git a/tests/plugins/test_goodgame.py b/tests/plugins/test_goodgame.py
--- a/tests/plugins/test_goodgame.py
+++ b/tests/plugins/test_goodgame.py
@@ -1,7 +1,4 @@
-import pytest
-
from streamlink.plugins.goodgame import GoodGame
-from streamlink.session import Streamlink
from tests.plugins import PluginCanHandleUrl
@@ -9,15 +6,21 @@ class TestPluginCanHandleUrlGoodGame(PluginCanHandleUrl):
__plugin__ = GoodGame
should_match_groups = [
- ("https://goodgame.ru/CHANNELNAME", {"user": "CHANNELNAME"}),
- ("https://goodgame.ru/channel/CHANNELNAME", {"user": "CHANNELNAME"}),
- ]
+ (("default", "https://goodgame.ru/CHANNELNAME"), {"name": "CHANNELNAME"}),
+ (("default", "https://goodgame.ru/CHANNELNAME/"), {"name": "CHANNELNAME"}),
+ (("default", "https://goodgame.ru/CHANNELNAME?foo=bar"), {"name": "CHANNELNAME"}),
+ (("default", "https://www.goodgame.ru/CHANNELNAME"), {"name": "CHANNELNAME"}),
+ (("channel", "https://goodgame.ru/channel/CHANNELNAME"), {"channel": "CHANNELNAME"}),
+ (("channel", "https://goodgame.ru/channel/CHANNELNAME/"), {"channel": "CHANNELNAME"}),
+ (("channel", "https://goodgame.ru/channel/CHANNELNAME?foo=bar"), {"channel": "CHANNELNAME"}),
+ (("channel", "https://www.goodgame.ru/channel/CHANNELNAME"), {"channel": "CHANNELNAME"}),
[email protected](("url", "expected"), [
- ("https://goodgame.ru/CHANNELNAME", "https://goodgame.ru/CHANNELNAME"),
- ("https://goodgame.ru/channel/CHANNELNAME", "https://goodgame.ru/CHANNELNAME"),
-])
-def test_url_translation(session: Streamlink, url: str, expected: str):
- plugin = GoodGame(session, url)
- assert plugin.url == expected
+ (("player", "https://goodgame.ru/player?1234"), {"id": "1234"}),
+ (("player", "https://www.goodgame.ru/player?1234"), {"id": "1234"}),
+ ]
+
+ should_not_match = [
+ "https://goodgame.ru/channel",
+ "https://goodgame.ru/player",
+ ]
| plugins.goodgame: Handling player links
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
streamlink 6.2.1
### Description
It would be great to handle links like https://goodgame.ru/player?199287.
This link represents a *player in window* link class, that can be accessed by clicking the player button.

### Debug log
```text
[cli][debug] OS: Linux-6.1.55-1-lts-x86_64-with-glibc2.38
[cli][debug] Python: 3.11.5
[cli][debug] OpenSSL: OpenSSL 3.1.3 19 Sep 2023
[cli][debug] Streamlink: 6.2.1
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.7.22
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.2
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.19.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.28.2
[cli][debug] trio: 0.22.2
[cli][debug] trio-websocket: 0.11.1
[cli][debug] typing-extensions: 4.8.0
[cli][debug] urllib3: 1.26.15
[cli][debug] websocket-client: 1.6.3
[cli][debug] Arguments:
[cli][debug] url=https://goodgame.ru/player?199287
[cli][debug] --loglevel=debug
[cli][info] Found matching plugin goodgame for URL https://goodgame.ru/player?199287
error: Unable to validate response text: ValidationError(Pattern):
Type of None should be str or bytes, but is NoneType
```
| 2023-10-04T13:45:59 |
|
streamlink/streamlink | 5,591 | streamlink__streamlink-5591 | [
"5590"
] | 1d4e60fe3b3771bc33c6c6f6fc303f4ab85d8d46 | diff --git a/src/streamlink/plugins/twitch.py b/src/streamlink/plugins/twitch.py
--- a/src/streamlink/plugins/twitch.py
+++ b/src/streamlink/plugins/twitch.py
@@ -17,12 +17,15 @@
import logging
import re
import sys
+from contextlib import suppress
from datetime import datetime, timedelta
from json import dumps as json_dumps
from random import random
from typing import List, Mapping, NamedTuple, Optional, Tuple
from urllib.parse import urlparse
+from requests.exceptions import HTTPError
+
from streamlink.exceptions import NoStreamsError, PluginError
from streamlink.plugin import Plugin, pluginargument, pluginmatcher
from streamlink.plugin.api import validate
@@ -900,11 +903,22 @@ def _get_hls_streams(self, url, restricted_bitrates, **extra_params):
**extra_params,
)
except OSError as err:
- err = str(err)
- if "404 Client Error" in err or "Failed to parse playlist" in err:
+ # TODO: fix the "err" attribute set by HTTPSession.request()
+ orig = getattr(err, "err", None)
+ if isinstance(orig, HTTPError) and orig.response.status_code >= 400:
+ error = None
+ with suppress(PluginError):
+ error = validate.Schema(
+ validate.parse_json(),
+ [{
+ "type": "error",
+ "error": str,
+ }],
+ validate.get((0, "error")),
+ ).validate(orig.response.text)
+ log.error(error or "Could not access HLS playlist")
return
- else:
- raise PluginError(err) from err
+ raise PluginError(err) from err
for name in restricted_bitrates:
if name not in streams:
| diff --git a/tests/plugins/test_twitch.py b/tests/plugins/test_twitch.py
--- a/tests/plugins/test_twitch.py
+++ b/tests/plugins/test_twitch.py
@@ -1,4 +1,5 @@
import unittest
+from contextlib import nullcontext
from datetime import datetime, timedelta, timezone
from unittest.mock import MagicMock, Mock, call, patch
@@ -585,6 +586,60 @@ def test_failed_integrity_check(self, plugin: Twitch, mock: rm.Mocker):
assert headers["Client-Integrity"] == "client-integrity-token"
+class TestTwitchHLSMultivariantResponse:
+ @pytest.fixture()
+ def plugin(self, request: pytest.FixtureRequest, requests_mock: rm.Mocker, session: Streamlink):
+ requests_mock.get("mock://multivariant", **getattr(request, "param", {}))
+ return Twitch(session, "https://twitch.tv/channelname")
+
+ @pytest.mark.parametrize(("plugin", "raises", "streams", "log"), [
+ pytest.param(
+ {"text": "#EXTM3U\n"},
+ nullcontext(),
+ {},
+ [],
+ id="success",
+ ),
+ pytest.param(
+ {"text": "Not an HLS playlist"},
+ pytest.raises(PluginError),
+ {},
+ [],
+ id="invalid HLS playlist",
+ ),
+ pytest.param(
+ {
+ "status_code": 403,
+ "json": [{
+ "url": "mock://multivariant",
+ "error": "Content Restricted In Region",
+ "error_code": "content_geoblocked",
+ "type": "error",
+ }],
+ },
+ nullcontext(),
+ None,
+ [("streamlink.plugins.twitch", "error", "Content Restricted In Region")],
+ id="geo restriction",
+ ),
+ pytest.param(
+ {
+ "status_code": 404,
+ "text": "Not found",
+ },
+ nullcontext(),
+ None,
+ [("streamlink.plugins.twitch", "error", "Could not access HLS playlist")],
+ id="non-json error response",
+ ),
+ ], indirect=["plugin"])
+ def test_multivariant_response(self, caplog: pytest.LogCaptureFixture, plugin: Twitch, raises, streams, log):
+ caplog.set_level("error", "streamlink.plugins.twitch")
+ with raises:
+ assert plugin._get_hls_streams("mock://multivariant", []) == streams
+ assert [(record.name, record.levelname, record.message) for record in caplog.records] == log
+
+
class TestTwitchMetadata:
@pytest.fixture()
def metadata(self, request: pytest.FixtureRequest, session: Streamlink):
| plugins.twitch: TypeError: exception causes must derive from BaseException
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
streamlink 6.2.0
### Description
I'm attempting to get a link for the free and publicly available stream of Thursday Night Football on Amazon's Twitch channel (twitch.tv/primevideo) to add to my Channels DVR so I can watch as if it was a traditional broadcast. It doesn't appear to matter if the channel is live or not as attempting to query offline channels simply returns a `No playable streams found on this URL` message whereas this causes a crash.
### Debug log
```text
PS C:\Users\*user*> streamlink twitch.tv/primevideo best
[cli][info] Found matching plugin twitch for URL twitch.tv/primevideo
Traceback (most recent call last):
File "C:\Users\*user*\AppData\Local\Programs\Streamlink\pkgs\streamlink\plugins\twitch.py", line 894, in _get_hls_streams
streams = TwitchHLSStream.parse_variant_playlist(
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "C:\Users\*user*\AppData\Local\Programs\Streamlink\pkgs\streamlink\stream\hls.py", line 710, in parse_variant_playlist
res = cls._fetch_variant_playlist(session, url, **request_args)
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "C:\Users\*user*\AppData\Local\Programs\Streamlink\pkgs\streamlink\stream\hls.py", line 666, in _fetch_variant_playlist
res = session.http.get(url, exception=OSError, **request_args)
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "C:\Users\*user*\AppData\Local\Programs\Streamlink\pkgs\requests\sessions.py", line 602, in get
return self.request("GET", url, **kwargs)
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "C:\Users\*user*\AppData\Local\Programs\Streamlink\pkgs\streamlink\plugin\api\http_session.py", line 190, in request
raise err from None # TODO: fix this
^^^^^^^^^^^^^^^^^^^
OSError: Unable to open URL: https://usher.ttvnw.net/api/channel/hls/primevideo.m3u8?player=twitchweb&p=358891&type=any&allow_source=true&allow_audio_only=true&allow_spectre=false&sig=f73c97aef3f78f0a09540d793663adcf94cdacfe&token=%7B%22adblock%22%3Afalse%2C%22authorization%22%3A%7B%22forbidden%22%3Afalse%2C%22reason%22%3A%22%22%7D%2C%22blackout_enabled%22%3Afalse%2C%22channel%22%3A%22primevideo%22%2C%22channel_id%22%3A168843586%2C%22chansub%22%3A%7B%22restricted_bitrates%22%3A%5B%5D%2C%22view_until%22%3A1924905600%7D%2C%22ci_gb%22%3Afalse%2C%22geoblock_reason%22%3A%22%22%2C%22device_id%22%3Anull%2C%22expires%22%3A1696547649%2C%22extended_history_allowed%22%3Afalse%2C%22game%22%3A%22%22%2C%22hide_ads%22%3Afalse%2C%22https_required%22%3Atrue%2C%22mature%22%3Afalse%2C%22partner%22%3Afalse%2C%22platform%22%3A%22web%22%2C%22player_type%22%3A%22embed%22%2C%22private%22%3A%7B%22allowed_to_view%22%3Atrue%7D%2C%22privileged%22%3Afalse%2C%22role%22%3A%22%22%2C%22server_ads%22%3Atrue%2C%22show_ads%22%3Atrue%2C%22subscriber%22%3Afalse%2C%22turbo%22%3Afalse%2C%22user_id%22%3Anull%2C%22user_ip%22%3A%2273.197.231.235%22%2C%22version%22%3A2%7D&fast_bread=True (403 Client Error: Forbidden for url: https://usher.ttvnw.net/api/channel/hls/primevideo.m3u8?player=twitchweb&p=358891&type=any&allow_source=true&allow_audio_only=true&allow_spectre=false&sig=f73c97aef3f78f0a09540d793663adcf94cdacfe&token=%7B%22adblock%22%3Afalse%2C%22authorization%22%3A%7B%22forbidden%22%3Afalse%2C%22reason%22%3A%22%22%7D%2C%22blackout_enabled%22%3Afalse%2C%22channel%22%3A%22primevideo%22%2C%22channel_id%22%3A168843586%2C%22chansub%22%3A%7B%22restricted_bitrates%22%3A%5B%5D%2C%22view_until%22%3A1924905600%7D%2C%22ci_gb%22%3Afalse%2C%22geoblock_reason%22%3A%22%22%2C%22device_id%22%3Anull%2C%22expires%22%3A1696547649%2C%22extended_history_allowed%22%3Afalse%2C%22game%22%3A%22%22%2C%22hide_ads%22%3Afalse%2C%22https_required%22%3Atrue%2C%22mature%22%3Afalse%2C%22partner%22%3Afalse%2C%22platform%22%3A%22web%22%2C%22player_type%22%3A%22embed%22%2C%22private%22%3A%7B%22allowed_to_view%22%3Atrue%7D%2C%22privileged%22%3Afalse%2C%22role%22%3A%22%22%2C%22server_ads%22%3Atrue%2C%22show_ads%22%3Atrue%2C%22subscriber%22%3Afalse%2C%22turbo%22%3Afalse%2C%22user_id%22%3Anull%2C%22user_ip%22%3A%2273.197.231.235%22%2C%22version%22%3A2%7D&fast_bread=True)
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "<frozen runpy>", line 198, in _run_module_as_main
File "<frozen runpy>", line 88, in _run_code
File "C:\Users\*user*\AppData\Local\Programs\Streamlink\bin\streamlink.exe\__main__.py", line 18, in <module>
File "C:\Users\*user*\AppData\Local\Programs\Streamlink\pkgs\streamlink_cli\main.py", line 929, in main
handle_url()
File "C:\Users\*user*\AppData\Local\Programs\Streamlink\pkgs\streamlink_cli\main.py", line 551, in handle_url
streams = fetch_streams(plugin)
^^^^^^^^^^^^^^^^^^^^^
File "C:\Users\*user*\AppData\Local\Programs\Streamlink\pkgs\streamlink_cli\main.py", line 449, in fetch_streams
return plugin.streams(stream_types=args.stream_types,
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "C:\Users\*user*\AppData\Local\Programs\Streamlink\pkgs\streamlink\plugin\plugin.py", line 366, in streams
ostreams = self._get_streams()
^^^^^^^^^^^^^^^^^^^
File "C:\Users\*user*\AppData\Local\Programs\Streamlink\pkgs\streamlink\plugins\twitch.py", line 930, in _get_streams
return self._get_hls_streams_live()
^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "C:\Users\*user*\AppData\Local\Programs\Streamlink\pkgs\streamlink\plugins\twitch.py", line 875, in _get_hls_streams_live
return self._get_hls_streams(url, restricted_bitrates)
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "C:\Users\*user*\AppData\Local\Programs\Streamlink\pkgs\streamlink\plugins\twitch.py", line 907, in _get_hls_streams
raise PluginError(err) from err
TypeError: exception causes must derive from BaseException
PS C:\Users\*user*> streamlink twitch.tv/primevideo --disable-ads
usage: streamlink [OPTIONS] <URL> [STREAM]
streamlink: error: unrecognized arguments: --disable-ads
PS C:\Users\*user*> streamlink twitch.tv/primevideo --twitch-disable-ads
[cli][info] Found matching plugin twitch for URL twitch.tv/primevideo
Traceback (most recent call last):
File "C:\Users\*user*\AppData\Local\Programs\Streamlink\pkgs\streamlink\plugins\twitch.py", line 894, in _get_hls_streams
streams = TwitchHLSStream.parse_variant_playlist(
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "C:\Users\*user*\AppData\Local\Programs\Streamlink\pkgs\streamlink\stream\hls.py", line 710, in parse_variant_playlist
res = cls._fetch_variant_playlist(session, url, **request_args)
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "C:\Users\*user*\AppData\Local\Programs\Streamlink\pkgs\streamlink\stream\hls.py", line 666, in _fetch_variant_playlist
res = session.http.get(url, exception=OSError, **request_args)
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "C:\Users\*user*\AppData\Local\Programs\Streamlink\pkgs\requests\sessions.py", line 602, in get
return self.request("GET", url, **kwargs)
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "C:\Users\*user*\AppData\Local\Programs\Streamlink\pkgs\streamlink\plugin\api\http_session.py", line 190, in request
raise err from None # TODO: fix this
^^^^^^^^^^^^^^^^^^^
OSError: Unable to open URL: https://usher.ttvnw.net/api/channel/hls/primevideo.m3u8?player=twitchweb&p=545759&type=any&allow_source=true&allow_audio_only=true&allow_spectre=false&sig=3fafdeab499760891161dd22b67516af6b183667&token=%7B%22adblock%22%3Afalse%2C%22authorization%22%3A%7B%22forbidden%22%3Afalse%2C%22reason%22%3A%22%22%7D%2C%22blackout_enabled%22%3Afalse%2C%22channel%22%3A%22primevideo%22%2C%22channel_id%22%3A168843586%2C%22chansub%22%3A%7B%22restricted_bitrates%22%3A%5B%5D%2C%22view_until%22%3A1924905600%7D%2C%22ci_gb%22%3Afalse%2C%22geoblock_reason%22%3A%22%22%2C%22device_id%22%3Anull%2C%22expires%22%3A1696547741%2C%22extended_history_allowed%22%3Afalse%2C%22game%22%3A%22%22%2C%22hide_ads%22%3Afalse%2C%22https_required%22%3Atrue%2C%22mature%22%3Afalse%2C%22partner%22%3Afalse%2C%22platform%22%3A%22web%22%2C%22player_type%22%3A%22embed%22%2C%22private%22%3A%7B%22allowed_to_view%22%3Atrue%7D%2C%22privileged%22%3Afalse%2C%22role%22%3A%22%22%2C%22server_ads%22%3Atrue%2C%22show_ads%22%3Atrue%2C%22subscriber%22%3Afalse%2C%22turbo%22%3Afalse%2C%22user_id%22%3Anull%2C%22user_ip%22%3A%2273.197.231.235%22%2C%22version%22%3A2%7D&fast_bread=True (403 Client Error: Forbidden for url: https://usher.ttvnw.net/api/channel/hls/primevideo.m3u8?player=twitchweb&p=545759&type=any&allow_source=true&allow_audio_only=true&allow_spectre=false&sig=3fafdeab499760891161dd22b67516af6b183667&token=%7B%22adblock%22%3Afalse%2C%22authorization%22%3A%7B%22forbidden%22%3Afalse%2C%22reason%22%3A%22%22%7D%2C%22blackout_enabled%22%3Afalse%2C%22channel%22%3A%22primevideo%22%2C%22channel_id%22%3A168843586%2C%22chansub%22%3A%7B%22restricted_bitrates%22%3A%5B%5D%2C%22view_until%22%3A1924905600%7D%2C%22ci_gb%22%3Afalse%2C%22geoblock_reason%22%3A%22%22%2C%22device_id%22%3Anull%2C%22expires%22%3A1696547741%2C%22extended_history_allowed%22%3Afalse%2C%22game%22%3A%22%22%2C%22hide_ads%22%3Afalse%2C%22https_required%22%3Atrue%2C%22mature%22%3Afalse%2C%22partner%22%3Afalse%2C%22platform%22%3A%22web%22%2C%22player_type%22%3A%22embed%22%2C%22private%22%3A%7B%22allowed_to_view%22%3Atrue%7D%2C%22privileged%22%3Afalse%2C%22role%22%3A%22%22%2C%22server_ads%22%3Atrue%2C%22show_ads%22%3Atrue%2C%22subscriber%22%3Afalse%2C%22turbo%22%3Afalse%2C%22user_id%22%3Anull%2C%22user_ip%22%3A%2273.197.231.235%22%2C%22version%22%3A2%7D&fast_bread=True)
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "<frozen runpy>", line 198, in _run_module_as_main
PS C:\Users\*user*> streamlink twitch.tv/primevideo best
[cli][info] Found matching plugin twitch for URL twitch.tv/primevideo
Traceback (most recent call last):
File "C:\Users\*user*\AppData\Local\Programs\Streamlink\pkgs\streamlink\plugins\twitch.py", line 894, in _get_hls_streams
streams = TwitchHLSStream.parse_variant_playlist(
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "C:\Users\*user*\AppData\Local\Programs\Streamlink\pkgs\streamlink\stream\hls.py", line 710, in parse_variant_playlist
res = cls._fetch_variant_playlist(session, url, **request_args)
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "C:\Users\*user*\AppData\Local\Programs\Streamlink\pkgs\streamlink\stream\hls.py", line 666, in _fetch_variant_playlist
res = session.http.get(url, exception=OSError, **request_args)
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "C:\Users\*user*\AppData\Local\Programs\Streamlink\pkgs\requests\sessions.py", line 602, in get
return self.request("GET", url, **kwargs)
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "C:\Users\*user*\AppData\Local\Programs\Streamlink\pkgs\streamlink\plugin\api\http_session.py", line 190, in request
raise err from None # TODO: fix this
^^^^^^^^^^^^^^^^^^^
OSError: Unable to open URL: https://usher.ttvnw.net/api/channel/hls/primevideo.m3u8?player=twitchweb&p=686255&type=any&allow_source=true&allow_audio_only=true&allow_spectre=false&sig=d4cbfd5096874231459d870a473420927e28f7c3&token=%7B%22adblock%22%3Afalse%2C%22authorization%22%3A%7B%22forbidden%22%3Afalse%2C%22reason%22%3A%22%22%7D%2C%22blackout_enabled%22%3Afalse%2C%22channel%22%3A%22primevideo%22%2C%22channel_id%22%3A168843586%2C%22chansub%22%3A%7B%22restricted_bitrates%22%3A%5B%5D%2C%22view_until%22%3A1924905600%7D%2C%22ci_gb%22%3Afalse%2C%22geoblock_reason%22%3A%22%22%2C%22device_id%22%3Anull%2C%22expires%22%3A1696547814%2C%22extended_history_allowed%22%3Afalse%2C%22game%22%3A%22%22%2C%22hide_ads%22%3Afalse%2C%22https_required%22%3Atrue%2C%22mature%22%3Afalse%2C%22partner%22%3Afalse%2C%22platform%22%3A%22web%22%2C%22player_type%22%3A%22embed%22%2C%22private%22%3A%7B%22allowed_to_view%22%3Atrue%7D%2C%22privileged%22%3Afalse%2C%22role%22%3A%22%22%2C%22server_ads%22%3Atrue%2C%22show_ads%22%3Atrue%2C%22subscriber%22%3Afalse%2C%22turbo%22%3Afalse%2C%22user_id%22%3Anull%2C%22user_ip%22%3A%2273.197.231.235%22%2C%22version%22%3A2%7D&fast_bread=True (403 Client Error: Forbidden for url: https://usher.ttvnw.net/api/channel/hls/primevideo.m3u8?player=twitchweb&p=686255&type=any&allow_source=true&allow_audio_only=true&allow_spectre=false&sig=d4cbfd5096874231459d870a473420927e28f7c3&token=%7B%22adblock%22%3Afalse%2C%22authorization%22%3A%7B%22forbidden%22%3Afalse%2C%22reason%22%3A%22%22%7D%2C%22blackout_enabled%22%3Afalse%2C%22channel%22%3A%22primevideo%22%2C%22channel_id%22%3A168843586%2C%22chansub%22%3A%7B%22restricted_bitrates%22%3A%5B%5D%2C%22view_until%22%3A1924905600%7D%2C%22ci_gb%22%3Afalse%2C%22geoblock_reason%22%3A%22%22%2C%22device_id%22%3Anull%2C%22expires%22%3A1696547814%2C%22extended_history_allowed%22%3Afalse%2C%22game%22%3A%22%22%2C%22hide_ads%22%3Afalse%2C%22https_required%22%3Atrue%2C%22mature%22%3Afalse%2C%22partner%22%3Afalse%2C%22platform%22%3A%22web%22%2C%22player_type%22%3A%22embed%22%2C%22private%22%3A%7B%22allowed_to_view%22%3Atrue%7D%2C%22privileged%22%3Afalse%2C%22role%22%3A%22%22%2C%22server_ads%22%3Atrue%2C%22show_ads%22%3Atrue%2C%22subscriber%22%3Afalse%2C%22turbo%22%3Afalse%2C%22user_id%22%3Anull%2C%22user_ip%22%3A%2273.197.231.235%22%2C%22version%22%3A2%7D&fast_bread=True)
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "<frozen runpy>", line 198, in _run_module_as_main
File "<frozen runpy>", line 88, in _run_code
File "C:\Users\*user*\AppData\Local\Programs\Streamlink\bin\streamlink.exe\__main__.py", line 18, in <module>
File "C:\Users\*user*\AppData\Local\Programs\Streamlink\pkgs\streamlink_cli\main.py", line 929, in main
handle_url()
File "C:\Users\*user*\AppData\Local\Programs\Streamlink\pkgs\streamlink_cli\main.py", line 551, in handle_url
streams = fetch_streams(plugin)
^^^^^^^^^^^^^^^^^^^^^
File "C:\Users\*user*\AppData\Local\Programs\Streamlink\pkgs\streamlink_cli\main.py", line 449, in fetch_streams
return plugin.streams(stream_types=args.stream_types,
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "C:\Users\*user*\AppData\Local\Programs\Streamlink\pkgs\streamlink\plugin\plugin.py", line 366, in streams
ostreams = self._get_streams()
^^^^^^^^^^^^^^^^^^^
File "C:\Users\*user*\AppData\Local\Programs\Streamlink\pkgs\streamlink\plugins\twitch.py", line 930, in _get_streams
return self._get_hls_streams_live()
^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "C:\Users\*user*\AppData\Local\Programs\Streamlink\pkgs\streamlink\plugins\twitch.py", line 875, in _get_hls_streams_live
return self._get_hls_streams(url, restricted_bitrates)
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "C:\Users\*user*\AppData\Local\Programs\Streamlink\pkgs\streamlink\plugins\twitch.py", line 907, in _get_hls_streams
raise PluginError(err) from err
TypeError: exception causes must derive from BaseException
```
| It's a 403 HTTP response on the HLS multivariant playlist request due to geo-restriction which isn't caught by the plugin (and its weird error handling). Let me fix this real quick... | 2023-10-06T00:20:51 |
streamlink/streamlink | 5,604 | streamlink__streamlink-5604 | [
"5602"
] | 0490c4eff16d3afad1566647a1eb3b91a1f4f59f | diff --git a/src/streamlink/plugins/ntv.py b/src/streamlink/plugins/ntv.py
deleted file mode 100644
--- a/src/streamlink/plugins/ntv.py
+++ /dev/null
@@ -1,31 +0,0 @@
-"""
-$description Russian live TV channel owned by Gazprom Media.
-$url ntv.ru
-$type live
-"""
-
-import re
-
-from streamlink.plugin import Plugin, pluginmatcher
-from streamlink.stream.hls import HLSStream
-
-
-@pluginmatcher(re.compile(
- r"https?://www\.ntv\.ru/air/",
-))
-class NTV(Plugin):
- def _get_streams(self):
- body = self.session.http.get(self.url).text
- mrl = None
- match = re.search(r"var camHlsURL = \'(.*)\'", body)
- if match:
- mrl = f"http:{match.group(1)}"
- else:
- match = re.search(r"var hlsURL = \'(.*)\'", body)
- if match:
- mrl = match.group(1)
- if mrl:
- return HLSStream.parse_variant_playlist(self.session, mrl)
-
-
-__plugin__ = NTV
| diff --git a/tests/plugins/test_ntv.py b/tests/plugins/test_ntv.py
deleted file mode 100644
--- a/tests/plugins/test_ntv.py
+++ /dev/null
@@ -1,16 +0,0 @@
-from streamlink.plugins.ntv import NTV
-from tests.plugins import PluginCanHandleUrl
-
-
-class TestPluginCanHandleUrlNTV(PluginCanHandleUrl):
- __plugin__ = NTV
-
- should_match = [
- "https://www.ntv.ru/air/",
- "http://www.ntv.ru/air/",
- ]
-
- should_not_match = [
- "https://www.ntv.ru/",
- "http://www.ntv.ru/",
- ]
| plugins.ntv: it have dead today
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
streamlink 6.2.1+23.ga39816fb
### Description
Yet yesterday it was working but now it has reached its end of life due to site changing. Fortunately, it works with static URL:
`http://cdn.ntv.ru/ntv-msk_hd/index.m3u8`
I have constructed this URL from very similar URLs taken from under the hood of the site.
So, we have 3 options:
1. Remove the plugin.
2. Mark it as broken.
3. Incorporate this static URL into plugin code as what to be returned.
I guess all of those decisions would be temporary.
### Debug log
```text
streamlink --loglevel=debug http://www.ntv.ru/air/
[cli][debug] OS: Linux-6.5.0-2-amd64-x86_64-with-glibc2.37
[cli][debug] Python: 3.11.6
[cli][debug] OpenSSL: OpenSSL 3.0.11 19 Sep 2023
[cli][debug] Streamlink: 6.2.1+23.ga39816fb
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.7.22
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.3
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.16.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.22.2
[cli][debug] trio-websocket: 0.10.3
[cli][debug] typing-extensions: 4.7.1
[cli][debug] urllib3: 1.26.17
[cli][debug] websocket-client: 1.2.3
[cli][debug] Arguments:
[cli][debug] url=http://www.ntv.ru/air/
[cli][debug] --loglevel=debug
[cli][debug] --player=mpv
[cli][debug] --player-args=--quiet
[cli][debug] --default-stream=['best']
[cli][info] Found matching plugin ntv for URL http://www.ntv.ru/air/
error: No playable streams found on this URL: http://www.ntv.ru/air/
```
| 2023-10-11T12:02:48 |
|
streamlink/streamlink | 5,607 | streamlink__streamlink-5607 | [
"5603"
] | e197337a0ddbfb77e7ecb045e76f583cb921bdc7 | diff --git a/src/streamlink/stream/hls/hls.py b/src/streamlink/stream/hls/hls.py
--- a/src/streamlink/stream/hls/hls.py
+++ b/src/streamlink/stream/hls/hls.py
@@ -462,8 +462,18 @@ def iter_segments(self):
continue
log.debug(f"Adding segment {segment.num} to queue")
- yield segment
+ offset = segment.num - self.playlist_sequence
+ if offset > 0:
+ log.warning(
+ (
+ f"Skipped segments {self.playlist_sequence}-{segment.num - 1} after playlist reload. "
+ if offset > 1 else
+ f"Skipped segment {self.playlist_sequence} after playlist reload. "
+ )
+ + "This is unsupported and will result in incoherent output data.",
+ )
+ yield segment
queued = True
total_duration += segment.duration
| diff --git a/tests/stream/hls/test_hls.py b/tests/stream/hls/test_hls.py
--- a/tests/stream/hls/test_hls.py
+++ b/tests/stream/hls/test_hls.py
@@ -198,6 +198,57 @@ def test_map(self):
assert self.called(map2, once=True), "Downloads second map only once"
+# TODO: finally rewrite the segmented/HLS test setup using pytest and replace redundant setups with parametrization
+@patch("streamlink.stream.hls.hls.HLSStreamWorker.wait", Mock(return_value=True))
+class TestHLSStreamPlaylistReloadDiscontinuity(TestMixinStreamHLS, unittest.TestCase):
+ @patch("streamlink.stream.hls.hls.log")
+ def test_no_discontinuity(self, mock_log: Mock):
+ thread, segments = self.subject([
+ Playlist(0, [Segment(0), Segment(1)]),
+ Playlist(2, [Segment(2), Segment(3)]),
+ Playlist(4, [Segment(4), Segment(5)], end=True),
+ ])
+
+ data = self.await_read(read_all=True)
+ assert data == self.content(segments)
+ assert all(self.called(s) for s in segments.values())
+ assert mock_log.warning.call_args_list == []
+
+ @patch("streamlink.stream.hls.hls.log")
+ def test_discontinuity_single_segment(self, mock_log: Mock):
+ thread, segments = self.subject([
+ Playlist(0, [Segment(0), Segment(1)]),
+ Playlist(2, [Segment(2), Segment(3)]),
+ Playlist(5, [Segment(5), Segment(6)]),
+ Playlist(8, [Segment(8), Segment(9)], end=True),
+ ])
+
+ data = self.await_read(read_all=True)
+ assert data == self.content(segments)
+ assert all(self.called(s) for s in segments.values())
+ assert mock_log.warning.call_args_list == [
+ call("Skipped segment 4 after playlist reload. This is unsupported and will result in incoherent output data."),
+ call("Skipped segment 7 after playlist reload. This is unsupported and will result in incoherent output data."),
+ ]
+
+ @patch("streamlink.stream.hls.hls.log")
+ def test_discontinuity_multiple_segments(self, mock_log: Mock):
+ thread, segments = self.subject([
+ Playlist(0, [Segment(0), Segment(1)]),
+ Playlist(2, [Segment(2), Segment(3)]),
+ Playlist(6, [Segment(6), Segment(7)]),
+ Playlist(10, [Segment(10), Segment(11)], end=True),
+ ])
+
+ data = self.await_read(read_all=True)
+ assert data == self.content(segments)
+ assert all(self.called(s) for s in segments.values())
+ assert mock_log.warning.call_args_list == [
+ call("Skipped segments 4-5 after playlist reload. This is unsupported and will result in incoherent output data."),
+ call("Skipped segments 8-9 after playlist reload. This is unsupported and will result in incoherent output data."),
+ ]
+
+
class TestHLSStreamWorker(TestMixinStreamHLS, unittest.TestCase):
__stream__ = EventedWorkerHLSStream
| stream.hls: Add warning when segments expire from playlist before being queued
### Checklist
- [X] This is a feature request and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed feature requests](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22feature+request%22)
### Description
Today my internet briefly went out while using streamlink to record an HLS stream, but came back up about a minute later. When it came back, it started downloading segments again, but I was surprised to see in the log that it simply skipped from segment 143 to 174 without any kind of log message indicating any issue. I had actually thought that streamlink output a warning when things like that occurred, but I realize now I was probably thinking of ffmpeg.
ffmpeg has a warning for when this situation occurs here:
https://github.com/FFmpeg/FFmpeg/blob/c06d3d24047206f9c11bfc5849544b960e5d68eb/libavformat/hls.c#L1518-L1523
It would be nice if streamlink could output a similar warning if it encounters non-consecutive sequence numbers. This wouldn't just be useful for internet issues but also potentially as an indicator that the `--hls-playlist-reload-time` needs to be set. I have occasionally encountered misbehaving HLS producers with wildly incorrect `EXT-X-TARGETDURATION` values causing segments to expire between playlist refreshes, but wouldn't necessarily have noticed any issue if I was just looking at the current log output.
| > it simply skipped from segment 143 to 174 without any kind of log message
Streamlink should log error messages on failed segment requests/resposes:
- https://github.com/streamlink/streamlink/blob/0490c4eff16d3afad1566647a1eb3b91a1f4f59f/src/streamlink/stream/hls/hls.py#L174-L182
- https://github.com/streamlink/streamlink/blob/0490c4eff16d3afad1566647a1eb3b91a1f4f59f/src/streamlink/stream/hls/hls.py#L270-L275
> When it came back, it started downloading segments again
HLS streams always continue despite segment errors, because it can be recovered from in most cases, even though it's a stream discontinuity. While this is bad for recording streams, it at least keeps live streams alive, which is the intention here and the main purpose of Streamlink. Recording is always an afterthought. Streamlink does only stop when the buffer timeout triggers (`--stream-timeout` - default is 60 seconds), meaning when the last `RingBuffer.read()` call times out due to missing data.
So if your connection came back before the stream timeout triggered, it should've logged error messages due to failed segment requests or failed playlist reloads. This of course depends on the timeout value you've set for individual HTTP requests (`--http-timeout` - default is 20 seconds). This affects both HTTP request connection timeouts and read timeouts for in-flight HTTP responses, but it's handled by `requests`, and not by Streamlink.
Did you change any HTTP options or the log output level?
Btw, annotated stream discontinuities get logged.
> Streamlink should log error messages on failed segment requests/resposes:
> Did you change any HTTP options or the log output level?
My options were
```
[cli][debug] --retry-streams=5.0
[cli][debug] --retry-max=12
[cli][debug] --retry-open=10
[cli][debug] --stream-segment-attempts=20
[cli][debug] --stream-segment-threads=10
[cli][debug] --stream-segment-timeout=60.0
[cli][debug] --stream-timeout=240.0
[cli][debug] --hls-playlist-reload-attempts=10
[cli][debug] --hls-playlist-reload-time=segment
[cli][debug] --hls-live-restart=True
```
and here's the relevant log section:
<details>
```
[02:56:08.176680][stream.hls][debug] Reloading playlist
[02:56:08.195682][stream.hls][debug] Adding segment 121 to queue
[02:56:08.243686][stream.hls][debug] Writing segment 121 to output
[02:56:08.245687][stream.hls][debug] Segment 121 complete
[02:56:10.164879][stream.hls][debug] Reloading playlist
[02:56:30.777982][stream.hls][debug] Adding segment 122 to queue
[02:56:30.777982][stream.hls][debug] Adding segment 123 to queue
[02:56:30.777982][stream.hls][debug] Adding segment 124 to queue
[02:56:30.778982][stream.hls][debug] Adding segment 125 to queue
[02:56:30.779983][stream.hls][debug] Adding segment 126 to queue
[02:56:30.783983][stream.hls][debug] Adding segment 127 to queue
[02:56:30.787983][stream.hls][debug] Adding segment 128 to queue
[02:56:30.791984][stream.hls][debug] Adding segment 129 to queue
[02:56:30.793984][stream.hls][debug] Adding segment 130 to queue
[02:56:30.799985][stream.hls][debug] Adding segment 131 to queue
[02:56:30.800985][stream.hls][debug] Adding segment 132 to queue
[02:56:30.801985][stream.hls][debug] Reloading playlist
[02:56:31.809098][stream.hls][debug] Reloading playlist
[02:56:33.339251][stream.hls][debug] Adding segment 133 to queue
[02:56:33.802297][stream.hls][debug] Reloading playlist
[02:56:33.911308][stream.hls][debug] Adding segment 134 to queue
[02:56:35.814500][stream.hls][debug] Reloading playlist
[02:56:35.901509][stream.hls][debug] Adding segment 135 to queue
[02:56:37.802699][stream.hls][debug] Reloading playlist
[02:56:37.982717][stream.hls][debug] Adding segment 136 to queue
[02:56:39.812900][stream.hls][debug] Reloading playlist
[02:56:40.122931][stream.hls][debug] Adding segment 137 to queue
[02:56:41.803099][stream.hls][debug] Reloading playlist
[02:56:41.924111][stream.hls][debug] Adding segment 138 to queue
[02:56:43.802299][stream.hls][debug] Reloading playlist
[02:56:43.863305][stream.hls][debug] Adding segment 139 to queue
[02:56:45.814500][stream.hls][debug] Reloading playlist
[02:56:45.966516][stream.hls][debug] Adding segment 140 to queue
[02:56:47.802699][stream.hls][debug] Reloading playlist
[02:56:47.892708][stream.hls][debug] Adding segment 141 to queue
[02:56:49.809900][stream.hls][debug] Reloading playlist
[02:56:49.953914][stream.hls][debug] Adding segment 142 to queue
[02:56:51.803099][stream.hls][debug] Reloading playlist
[02:56:52.116130][stream.hls][debug] Adding segment 143 to queue
[02:58:16.990940][stream.hls][debug] Writing segment 122 to output
[02:58:17.003443][stream.hls][debug] Segment 122 complete
[02:58:17.003943][stream.hls][debug] Writing segment 123 to output
[02:58:17.007944][stream.hls][debug] Segment 123 complete
[02:58:17.008944][stream.hls][debug] Reloading playlist
[02:58:17.323004][stream.hls][debug] Adding segment 172 to queue
[02:58:17.323505][stream.hls][debug] Adding segment 173 to queue
[02:58:18.589744][stream.hls][debug] Writing segment 124 to output
[02:58:18.592745][stream.hls][debug] Segment 124 complete
[02:58:18.593745][stream.hls][debug] Writing segment 125 to output
[02:58:18.598746][stream.hls][debug] Segment 125 complete
[02:58:18.599246][stream.hls][debug] Writing segment 126 to output
[02:58:18.599246][stream.hls][debug] Adding segment 174 to queue
[02:58:18.626752][stream.hls][debug] Segment 126 complete
[02:58:18.654257][stream.hls][debug] Writing segment 127 to output
[02:58:18.650757][stream.hls][debug] Adding segment 175 to queue
[02:58:18.660258][stream.hls][debug] Segment 127 complete
[02:58:18.675761][stream.hls][debug] Writing segment 128 to output
[02:58:18.675261][stream.hls][debug] Adding segment 176 to queue
[02:58:18.679762][stream.hls][debug] Segment 128 complete
[02:58:18.733273][stream.hls][debug] Adding segment 177 to queue
[02:58:18.733273][stream.hls][debug] Writing segment 129 to output
[02:58:18.741275][stream.hls][debug] Adding segment 178 to queue
[02:58:18.745776][stream.hls][debug] Segment 129 complete
[02:58:18.753277][stream.hls][debug] Writing segment 130 to output
[02:58:18.754777][stream.hls][debug] Segment 130 complete
[02:58:18.756778][stream.hls][debug] Writing segment 131 to output
[02:58:18.759278][stream.hls][debug] Segment 131 complete
[02:58:18.759778][stream.hls][debug] Adding segment 179 to queue
[02:58:18.825292][stream.hls][debug] Adding segment 180 to queue
[02:58:18.825292][stream.hls][debug] Adding segment 181 to queue
[02:58:18.760278][stream.hls][debug] Writing segment 132 to output
[02:58:18.831293][stream.hls][debug] Segment 132 complete
[02:58:18.838794][stream.hls][debug] Writing segment 133 to output
[02:58:18.842795][stream.hls][debug] Segment 133 complete
[02:58:18.843795][stream.hls][debug] Adding segment 182 to queue
[02:58:18.846796][stream.hls][debug] Writing segment 134 to output
[02:58:18.847296][stream.hls][debug] Adding segment 183 to queue
[02:58:18.850296][stream.hls][debug] Segment 134 complete
[02:58:18.876802][stream.hls][debug] Writing segment 135 to output
[02:58:18.883303][stream.hls][debug] Adding segment 184 to queue
[02:58:18.887804][stream.hls][debug] Segment 135 complete
[02:58:18.888804][stream.hls][debug] Writing segment 136 to output
[02:58:18.891805][stream.hls][debug] Segment 136 complete
[02:58:18.892805][stream.hls][debug] Writing segment 137 to output
[02:58:18.899306][stream.hls][debug] Segment 137 complete
[02:58:18.899306][stream.hls][debug] Writing segment 138 to output
[02:58:18.912309][stream.hls][debug] Segment 138 complete
[02:58:18.912809][stream.hls][debug] Adding segment 185 to queue
[02:58:18.912809][stream.hls][debug] Writing segment 139 to output
[02:58:18.938314][stream.hls][debug] Segment 139 complete
[02:58:18.938814][stream.hls][debug] Writing segment 140 to output
[02:58:18.941314][stream.hls][debug] Segment 140 complete
[02:58:18.943815][stream.hls][debug] Writing segment 141 to output
[02:58:18.948316][stream.hls][debug] Segment 141 complete
[02:58:18.951317][stream.hls][debug] Writing segment 142 to output
[02:58:18.953817][stream.hls][debug] Segment 142 complete
[02:58:18.954317][stream.hls][debug] Writing segment 143 to output
[02:58:18.957318][stream.hls][debug] Segment 143 complete
[02:58:18.958318][stream.hls][debug] Writing segment 172 to output
[02:58:18.963819][stream.hls][debug] Segment 172 complete
[02:58:19.066339][stream.hls][debug] Reloading playlist
```
</details>
So all the queued segments actually completed fine eventually (within the timeout period), there was just a period where the playlist request hung (due to my internet going out), and during that period obviously the playlist segments keep getting appended and removed, so when the request finally went through, a lot of segments were "missed". It's those "missed" segments that I'm requesting a warning about, similar to the ffmpeg one.
For example, for this case, streamlink did a playlist refresh and saw a maximum segment number of `143`, then in the next playlist refresh (that happened to be much later than expected because of network issues), the lowest segment number was `172`. If the lowest segment number of the new playlist is greater than the maximum of the old playlist + 1, then we've "dropped" segments between refreshes. This is what I want a warning about. If we go based on the ffmpeg warning, it would be `[warning] Skipping 28 segments ahead, expired from playlists` in this case. Personally I don't really like that warning, I think it could be more descriptive, but just some kind of indicator that segments were missed between playlist refreshes would be nice.
We can add such a warning, similar to the warning of the annotated discontinuities. | 2023-10-12T12:19:03 |
streamlink/streamlink | 5,610 | streamlink__streamlink-5610 | [
"5608"
] | 1f9a0e4191d7ca6f91404b87df3a7202a0047d81 | diff --git a/src/streamlink/stream/dash/manifest.py b/src/streamlink/stream/dash/manifest.py
--- a/src/streamlink/stream/dash/manifest.py
+++ b/src/streamlink/stream/dash/manifest.py
@@ -77,8 +77,14 @@ def type(mpdtype: Literal["static", "dynamic"]) -> Literal["static", "dynamic"]:
return mpdtype
@staticmethod
- def duration(duration: str) -> Union[timedelta, Duration]:
- return parse_duration(duration)
+ def duration(anchor: Optional[datetime] = None) -> Callable[[str], timedelta]:
+ def duration_to_timedelta(duration: str) -> timedelta:
+ parsed: Union[timedelta, Duration] = parse_duration(duration)
+ if isinstance(parsed, Duration):
+ return parsed.totimedelta(start=anchor or now())
+ return parsed
+
+ return duration_to_timedelta
@staticmethod
def datetime(dt: str) -> datetime:
@@ -286,6 +292,8 @@ class MPD(MPDNode):
parent: None # type: ignore[assignment]
timelines: Dict[TTimelineIdent, int]
+ DEFAULT_MINBUFFERTIME = 3.0
+
def __init__(self, *args, url: Optional[str] = None, **kwargs) -> None:
# top level has no parent
kwargs["root"] = self
@@ -307,19 +315,10 @@ def __init__(self, *args, url: Optional[str] = None, **kwargs) -> None:
parser=MPDParsers.type,
default="static",
)
- self.minimumUpdatePeriod = self.attr(
- "minimumUpdatePeriod",
- parser=MPDParsers.duration,
- default=timedelta(),
- )
- self.minBufferTime: Union[timedelta, Duration] = self.attr(
- "minBufferTime",
- parser=MPDParsers.duration,
- required=True,
- )
- self.timeShiftBufferDepth = self.attr(
- "timeShiftBufferDepth",
- parser=MPDParsers.duration,
+ self.publishTime = self.attr(
+ "publishTime",
+ parser=MPDParsers.datetime,
+ required=self.type == "dynamic",
)
self.availabilityStartTime = self.attr(
"availabilityStartTime",
@@ -327,26 +326,38 @@ def __init__(self, *args, url: Optional[str] = None, **kwargs) -> None:
default=EPOCH_START,
required=self.type == "dynamic",
)
- self.publishTime = self.attr(
- "publishTime",
- parser=MPDParsers.datetime,
- required=self.type == "dynamic",
- )
self.availabilityEndTime = self.attr(
"availabilityEndTime",
parser=MPDParsers.datetime,
)
+ self.minBufferTime: timedelta = self.attr( # type: ignore[assignment]
+ "minBufferTime",
+ parser=MPDParsers.duration(self.publishTime),
+ required=True,
+ )
+ self.minimumUpdatePeriod = self.attr(
+ "minimumUpdatePeriod",
+ parser=MPDParsers.duration(self.publishTime),
+ default=timedelta(),
+ )
+ self.timeShiftBufferDepth = self.attr(
+ "timeShiftBufferDepth",
+ parser=MPDParsers.duration(self.publishTime),
+ )
self.mediaPresentationDuration = self.attr(
"mediaPresentationDuration",
- parser=MPDParsers.duration,
+ parser=MPDParsers.duration(self.publishTime),
default=timedelta(),
)
self.suggestedPresentationDelay = self.attr(
"suggestedPresentationDelay",
- parser=MPDParsers.duration,
- # if there is no delay, use a delay of 3 seconds
+ parser=MPDParsers.duration(self.publishTime),
+ # if there is no delay, use a delay of 3 seconds, but respect the manifest's minBufferTime
# TODO: add a customizable parameter for this
- default=timedelta(seconds=3),
+ default=timedelta(seconds=max(
+ self.DEFAULT_MINBUFFERTIME,
+ self.minBufferTime.total_seconds(),
+ )),
)
# parse children
@@ -423,12 +434,12 @@ def __init__(self, *args, **kwargs) -> None:
)
self.duration = self.attr(
"duration",
- parser=MPDParsers.duration,
+ parser=MPDParsers.duration(self.root.publishTime),
default=timedelta(),
)
self.start = self.attr(
"start",
- parser=MPDParsers.duration,
+ parser=MPDParsers.duration(self.root.publishTime),
default=timedelta(),
)
| diff --git a/tests/resources/dash/test_suggested_presentation_delay.mpd b/tests/resources/dash/test_suggested_presentation_delay.mpd
new file mode 100644
--- /dev/null
+++ b/tests/resources/dash/test_suggested_presentation_delay.mpd
@@ -0,0 +1,27 @@
+<?xml version="1.0" encoding="utf-8"?>
+<MPD
+ xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
+ xmlns="urn:mpeg:dash:schema:mpd:2011"
+ xsi:schemaLocation="urn:mpeg:dash:schema:mpd:2011 DASH-MPD.xsd"
+ profiles="urn:mpeg:dash:profile:isoff-live:2011,http://dashif.org/guidelines/dash-if-simple"
+ type="dynamic"
+ availabilityStartTime="1970-01-01T00:00:00Z"
+ publishTime="2000-01-01T00:00:00Z"
+ maxSegmentDuration="PT5S"
+ minBufferTime="PT5S"
+>
+ <Period id="p0" start="PT0S">
+ <AdaptationSet mimeType="video/mp4" segmentAlignment="true" startWithSAP="1">
+ <SegmentTemplate initialization="$RepresentationID$_init.mp4" media="$RepresentationID$_t$Time$.m4s" timescale="90000">
+ <SegmentTimeline>
+ <S t="152740179303376" d="360000" r="899"/>
+ </SegmentTimeline>
+ </SegmentTemplate>
+ <Representation id="V0" bandwidth="1255000" codecs="avc1.640028" frameRate="25" width="1920" height="1080" sar="1:1"/>
+ <Representation id="V1" bandwidth="535000" codecs="avc1.64001f" frameRate="25" width="1280" height="720" sar="1:1"/>
+ <Representation id="V2" bandwidth="353000" codecs="avc1.4d401f" frameRate="25" width="960" height="540" sar="1:1"/>
+ <Representation id="V3" bandwidth="213000" codecs="avc1.4d401e" frameRate="25" width="768" height="432" sar="1:1"/>
+ <Representation id="V4" bandwidth="157000" codecs="avc1.4d401e" frameRate="25" width="640" height="360" sar="1:1"/>
+ </AdaptationSet>
+ </Period>
+</MPD>
diff --git a/tests/stream/dash/test_manifest.py b/tests/stream/dash/test_manifest.py
--- a/tests/stream/dash/test_manifest.py
+++ b/tests/stream/dash/test_manifest.py
@@ -7,9 +7,11 @@
from freezegun import freeze_time
from streamlink.stream.dash.manifest import MPD, DASHSegment, MPDParsers, MPDParsingError, Representation
+from streamlink.utils.times import fromtimestamp
from tests.resources import xml
+EPOCH_START = fromtimestamp(0)
UTC = datetime.timezone.utc
@@ -69,10 +71,22 @@ def test_type(self):
assert MPDParsers.type("dynamic") == "dynamic"
assert MPDParsers.type("static") == "static"
with pytest.raises(MPDParsingError):
+ # noinspection PyTypeChecker
MPDParsers.type("other")
def test_duration(self):
- assert MPDParsers.duration("PT1S") == datetime.timedelta(0, 1)
+ assert MPDParsers.duration()("PT1S") == datetime.timedelta(seconds=1)
+ assert MPDParsers.duration()("P1W") == datetime.timedelta(seconds=7 * 24 * 3600)
+
+ assert MPDParsers.duration(EPOCH_START)("P3M") == datetime.timedelta(days=31 + 28 + 31)
+ assert MPDParsers.duration(EPOCH_START + datetime.timedelta(31))("P3M") == datetime.timedelta(days=28 + 31 + 30)
+
+ assert MPDParsers.duration(EPOCH_START)("P3Y") == datetime.timedelta(days=3 * 365 + 1)
+ assert MPDParsers.duration(EPOCH_START + datetime.timedelta(3 * 365))("P3Y") == datetime.timedelta(days=3 * 365)
+
+ with freeze_time(EPOCH_START):
+ assert MPDParsers.duration()("P3M") == datetime.timedelta(days=31 + 28 + 31)
+ assert MPDParsers.duration()("P3Y") == datetime.timedelta(days=3 * 365 + 1)
def test_datetime(self):
assert MPDParsers.datetime("2018-01-01T00:00:00Z") == datetime.datetime(2018, 1, 1, 0, 0, 0, tzinfo=UTC)
@@ -98,6 +112,16 @@ def test_range(self):
class TestMPDParser:
+ @pytest.mark.parametrize(("min_buffer_time", "expected"), [
+ pytest.param("PT1S", 3.0, id="minBufferTime lower than suggestedPresentationDelay"),
+ pytest.param("PT5S", 5.0, id="minBufferTime greater than suggestedPresentationDelay"),
+ ])
+ def test_suggested_presentation_delay(self, min_buffer_time: str, expected: float):
+ with xml("dash/test_suggested_presentation_delay.mpd") as mpd_xml:
+ mpd_xml.attrib["minBufferTime"] = min_buffer_time
+ mpd = MPD(mpd_xml, base_url="http://test/", url="http://test/manifest.mpd")
+ assert mpd.suggestedPresentationDelay.total_seconds() == expected
+
def test_no_segment_list_or_template(self):
with xml("dash/test_no_segment_list_or_template.mpd") as mpd_xml:
mpd = MPD(mpd_xml, base_url="http://test/", url="http://test/manifest.mpd")
| stream.dash: default suggestedPresentationDelay shouldn't be below minBufferTime
### Checklist
- [ ] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
6.2.1
### Description
I have a MPD without a suggestedPresentationDelay, so it defaults to 3 seconds. The current code starts playback, but after a few seconds, it's halted again, to continue after a few seconds.
I'm not 100% sure how the `segment_timeline` method works, but it seems that the last possible segment is selected, and a new segment isn't available in time, causing the stream to hang for a few seconds after the first segment has played fine. Writing the stream to a file and playing that works fine.
When I manually modify the `suggestedPresentationDelay` to 10, there is no issue. Setting it to 5 can sometimes still cause the stream to hang at the beginning.
```py
self.suggestedPresentationDelay = self.attr(
"suggestedPresentationDelay",
parser=MPDParsers.duration,
# if there is no delay, use a delay of 3 seconds
# TODO: add a customizable parameter for this
default=timedelta(seconds=10),
)
```
I'm wondering if the `suggestedPresentationDelay` should be at least the value of minBufferTime if it's missing from the manifest? I guess that ideally, it shouldn't pick the latest segment, like with `--hls-live-edge` for HLS streams.
Manifest with some stripped `<S>` tags to decrease the size.
```xml
<?xml version="1.0" encoding="utf-8"?>
<MPD
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="urn:mpeg:dash:schema:mpd:2011"
xmlns:ns1="http://www.w3.org/2001/XMLSchema-instance"
xmlns:cenc="urn:mpeg:cenc:2013"
xsi:schemaLocation="urn:mpeg:dash:schema:mpd:2011 DASH-MPD.xsd"
profiles="urn:mpeg:dash:profile:isoff-live:2011,http://dashif.org/guidelines/dash-if-simple"
maxSegmentDuration="PT5S" minBufferTime="PT5S" type="dynamic" publishTime="2023-10-12T13:18:26Z" timeShiftBufferDepth="PT1H0S" availabilityStartTime="1970-01-01T00:00:00Z" minimumUpdatePeriod="PT0S">
<Period id="p0" start="PT0S">
<AdaptationSet mimeType="video/mp4" segmentAlignment="true" startWithSAP="1">
<SegmentTemplate initialization="sc-gaFECw/$RepresentationID$_init.mp4" media="sc-gaFECw/$RepresentationID$_t$Time$.m4s" timescale="90000">
<SegmentTimeline>
<S t="152740179303376" d="360000" r="899"/>
</SegmentTimeline>
</SegmentTemplate>
<Representation id="V0" bandwidth="1255000" codecs="avc1.640028" frameRate="25" width="1920" height="1080" sar="1:1"/>
<Representation id="V1" bandwidth="535000" codecs="avc1.64001f" frameRate="25" width="1280" height="720" sar="1:1"/>
<Representation id="V2" bandwidth="353000" codecs="avc1.4d401f" frameRate="25" width="960" height="540" sar="1:1"/>
<Representation id="V3" bandwidth="213000" codecs="avc1.4d401e" frameRate="25" width="768" height="432" sar="1:1"/>
<Representation id="V4" bandwidth="157000" codecs="avc1.4d401e" frameRate="25" width="640" height="360" sar="1:1"/>
</AdaptationSet>
<AdaptationSet mimeType="audio/mp4" lang="nld" segmentAlignment="true" startWithSAP="1">
<Role schemeIdUri="urn:mpeg:dash:role:2011" value="main"/>
<SegmentTemplate initialization="sc-gaFECw/$RepresentationID$_init.mp4" media="sc-gaFECw/$RepresentationID$_t$Time$.m4s" timescale="90000">
<SegmentTimeline>
<S t="152740179304696" d="359040"/>
<S d="360960"/>
<S d="359040"/>
<S d="360960"/>
<S d="359040"/>
<S d="360960"/>
<!-- cut -->
<S d="360960"/>
<S d="359040"/>
<S d="360960"/>
</SegmentTimeline>
</SegmentTemplate>
<Representation id="A0" bandwidth="128000" codecs="mp4a.40.2" audioSamplingRate="48000"><AudioChannelConfiguration schemeIdUri="urn:mpeg:dash:23003:3:audio_channel_configuration:2011" value="2"/></Representation>
</AdaptationSet>
</Period>
</MPD>
```
### Debug log
```text
[cli][debug] OS: Linux-5.15.123.1-microsoft-standard-WSL2-x86_64-with-glibc2.35
[cli][debug] Python: 3.10.12
[cli][debug] OpenSSL: OpenSSL 3.0.2 15 Mar 2022
[cli][debug] Streamlink: 6.2.1+23.g0490c4ef.dirty
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.7.22
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.3
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.19.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.22.2
[cli][debug] trio-websocket: 0.11.1
[cli][debug] typing-extensions: 4.8.0
[cli][debug] urllib3: 2.0.6
[cli][debug] websocket-client: 1.6.4
[cli][debug] Arguments:
[cli][debug] url=https://xxx/dash.mpd
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --output=dump.ts
[cli][debug] --ffmpeg-fout=mpegts
[cli][info] Found matching plugin dash for URL https://xxx/dash.mpd
[plugins.dash][debug] URL=https://xxx/dash.mpd; params={}
[utils.l10n][debug] Language code: nl_BE
[stream.dash][debug] Available languages for DASH audio streams: nld (using: nld)
[cli][info] Available streams: 360p (worst), 432p, 540p, 720p, 1080p (best)
[cli][info] Opening stream: 1080p (dash)
[cli][info] Writing output to
/home/michael/Development/streamlink/dump.ts
[cli][debug] Checking file output
[stream.dash][debug] Opening DASH reader for: ('p0', None, 'V0') - video/mp4
[stream.dash][debug] video/mp4 segment initialization: downloading (1970-01-01T00:00:00.000000Z / 2023-10-12T13:34:03.535015Z)
[stream.dash.manifest][debug] Generating segment timeline for dynamic playlist: ('p0', None, 'V0')
[stream.dash][debug] Opening DASH reader for: ('p0', None, 'A0') - audio/mp4
[stream.dash][debug] Reloading manifest ('p0', None, 'V0')
[stream.dash][debug] audio/mp4 segment initialization: downloading (1970-01-01T00:00:00.000000Z / 2023-10-12T13:34:03.538269Z)
[stream.dash.manifest][debug] Generating segment timeline for dynamic playlist: ('p0', None, 'A0')
[stream.dash][debug] Reloading manifest ('p0', None, 'A0')
[stream.dash][debug] video/mp4 segment 900: waiting 0.4s (2023-10-12T13:34:04.000000Z / 2023-10-12T13:34:03.555758Z)
[stream.dash][debug] video/mp4 segment initialization: completed
[stream.ffmpegmux][debug] ffmpeg version 6.0 Copyright (c) 2000-2023 the FFmpeg developers
[stream.ffmpegmux][debug] built with gcc 11 (Ubuntu 11.3.0-1ubuntu1~22.04.1)
[stream.ffmpegmux][debug] configuration: --disable-decoder=amrnb --disable-decoder=libopenjpeg --disable-gnutls --disable-liblensfun --disable-libopencv --disable-podpages --disable-sndio --disable-stripping --enable-avfilter --enable-chromaprint --enable-frei0r --enable-gcrypt --enable-gpl --enable-ladspa --enable-libaom --enable-libaribb24 --enable-libass --enable-libbluray --enable-libbs2b --enable-libcaca --enable-libcdio --enable-libcodec2 --enable-libdav1d --enable-libdc1394 --enable-libdrm --enable-libfdk-aac --enable-libflite --enable-libfontconfig --enable-libfreetype --enable-libfribidi --enable-libglslang --enable-libgme --enable-libgsm --enable-libiec61883 --enable-libjack --enable-libkvazaar --enable-libmp3lame --enable-libmysofa --enable-libopencore-amrnb --enable-libopencore-amrwb --enable-libopenh264 --enable-libopenjpeg --enable-libopenmpt --enable-libopus --enable-libpulse --enable-librabbitmq --enable-librist --enable-librsvg --enable-librubberband --enable-libshine --enable-libsmbclient --enable-libsnappy --enable-libsoxr --enable-libspeex --enable-libsrt --enable-libsvtav1 --enable-libtesseract --enable-libtheora --enable-libtwolame --enable-libvidstab --enable-libvo-amrwbenc --enable-libvorbis --enable-libvpx --enable-libwebp --enable-libwebp --enable-libx264 --enable-libx265 --enable-libxml2 --enable-libxvid --enable-libzimg --enable-libzmq --enable-libzvbi --enable-lv2 --enable-nonfree --enable-omx --enable-openal --enable-opencl --enable-opengl --enable-openssl --enable-postproc --enable-pthreads --enable-shared --enable-version3 --enable-vulkan --incdir=/usr/include/x86_64-linux-gnu --libdir=/usr/lib/x86_64-linux-gnu --prefix=/usr --toolchain=hardened --enable-vaapi --enable-libvpl --enable-libvmaf --enable-libdavs2 --enable-libxavs2 --enable-libilbc --enable-libjxl --disable-altivec --shlibdir=/usr/lib/x86_64-linux-gnu
[stream.ffmpegmux][debug] libavutil 58. 2.100 / 58. 2.100
[stream.ffmpegmux][debug] libavcodec 60. 3.100 / 60. 3.100
[stream.ffmpegmux][debug] libavformat 60. 3.100 / 60. 3.100
[stream.ffmpegmux][debug] libavdevice 60. 1.100 / 60. 1.100
[stream.ffmpegmux][debug] libavfilter 9. 3.100 / 9. 3.100
[stream.ffmpegmux][debug] libswscale 7. 1.100 / 7. 1.100
[stream.ffmpegmux][debug] libswresample 4. 10.100 / 4. 10.100
[stream.ffmpegmux][debug] libpostproc 57. 1.100 / 57. 1.100
[utils.named_pipe][info] Creating pipe streamlinkpipe-66036-1-7821
[utils.named_pipe][info] Creating pipe streamlinkpipe-66036-2-9899
[stream.ffmpegmux][debug] ffmpeg command: /usr/bin/ffmpeg -nostats -y -i /tmp/streamlinkpipe-66036-1-7821 -i /tmp/streamlinkpipe-66036-2-9899 -c:v copy -c:a copy -copyts -f mpegts pipe:1
[stream.ffmpegmux][debug] Starting copy to pipe: /tmp/streamlinkpipe-66036-1-7821
[stream.ffmpegmux][debug] Starting copy to pipe: /tmp/streamlinkpipe-66036-2-9899
[cli][debug] Pre-buffering 8192 bytes
[stream.dash][debug] audio/mp4 segment 900: waiting 0.1s (2023-10-12T13:34:04.000000Z / 2023-10-12T13:34:03.912906Z)
[stream.dash][debug] audio/mp4 segment initialization: completed
[stream.dash][debug] video/mp4 segment 900: downloading (2023-10-12T13:34:04.000000Z / 2023-10-12T13:34:04.000367Z)
[stream.dash][debug] audio/mp4 segment 900: downloading (2023-10-12T13:34:04.000000Z / 2023-10-12T13:34:04.001298Z)
[stream.dash][debug] audio/mp4 segment 900: completed
[stream.dash][debug] video/mp4 segment 900: completed
[cli][debug] Writing stream to output
[download] Written 1.42 MiB to dump.ts (4s @ 387.32 KiB/s)
[stream.dash.manifest][debug] Generating segment timeline for dynamic playlist: ('p0', None, 'V0')
[stream.dash][debug] Reloading manifest ('p0', None, 'V0')
[stream.dash.manifest][debug] Generating segment timeline for dynamic playlist: ('p0', None, 'A0')
[stream.dash][debug] Reloading manifest ('p0', None, 'A0')
[download] Written 1.42 MiB to dump.ts (11s @ 127.71 KiB/s)
[stream.dash.manifest][debug] Generating segment timeline for dynamic playlist: ('p0', None, 'V0')
[stream.dash][debug] Reloading manifest ('p0', None, 'V0')
[stream.dash][debug] video/mp4 segment 900: downloading (2023-10-12T13:34:10.000000Z / 2023-10-12T13:34:16.157370Z)
[stream.dash.manifest][debug] Generating segment timeline for dynamic playlist: ('p0', None, 'A0')
[stream.dash][debug] Reloading manifest ('p0', None, 'A0')
[stream.dash][debug] audio/mp4 segment 900: downloading (2023-10-12T13:34:10.000000Z / 2023-10-12T13:34:16.165648Z)
[stream.dash][debug] audio/mp4 segment 900: completed
[download] Written 1.46 MiB to dump.ts (11s @ 129.09 KiB/s)
[stream.dash][debug] video/mp4 segment 900: completed
[download] Written 2.95 MiB to dump.ts (16s @ 184.29 KiB/s)
[stream.dash.manifest][debug] Generating segment timeline for dynamic playlist: ('p0', None, 'V0')
[stream.dash][debug] Reloading manifest ('p0', None, 'V0')
[stream.dash][debug] video/mp4 segment 899: downloading (2023-10-12T13:34:12.000000Z / 2023-10-12T13:34:21.157445Z)
[stream.dash.manifest][debug] Generating segment timeline for dynamic playlist: ('p0', None, 'A0')
[stream.dash][debug] Reloading manifest ('p0', None, 'A0')
[stream.dash][debug] audio/mp4 segment 899: downloading (2023-10-12T13:34:11.989333Z / 2023-10-12T13:34:21.162231Z)
[download] Written 2.95 MiB to dump.ts (16s @ 181.52 KiB/s)
[stream.dash][debug] video/mp4 segment 900: downloading (2023-10-12T13:34:16.000000Z / 2023-10-12T13:34:21.402217Z)
[stream.dash][debug] video/mp4 segment 899: completed
[stream.dash][debug] audio/mp4 segment 900: downloading (2023-10-12T13:34:16.000000Z / 2023-10-12T13:34:21.408679Z)
[stream.dash][debug] audio/mp4 segment 899: completed
[stream.dash][debug] audio/mp4 segment 900: completed
[download] Written 4.20 MiB to dump.ts (17s @ 254.53 KiB/s)
[stream.dash][debug] video/mp4 segment 900: completed
[download] Written 5.84 MiB to dump.ts (18s @ 334.09 KiB/s)
^C
[stream.ffmpegmux][debug] Closing ffmpeg thread
[stream.segmented][debug] Closing worker thread
[stream.segmented][debug] Closing writer thread
[stream.ffmpegmux][debug] Pipe copy complete: /tmp/streamlinkpipe-66036-1-7821
[stream.segmented][debug] Closing worker thread
[stream.segmented][debug] Closing writer thread
[stream.ffmpegmux][debug] Pipe copy complete: /tmp/streamlinkpipe-66036-2-9899
[stream.ffmpegmux][debug] Closed all the substreams
[cli][info] Stream ended
Interrupted! Exiting...
[cli][info] Closing currently open stream...
```
| > I'm not 100% sure how the `segment_timeline` method works, but it seems that the last possible segment is selected
It yields as many segments as needed until the threshold is reached.
https://github.com/streamlink/streamlink/blob/e197337a0ddbfb77e7ecb045e76f583cb921bdc7/src/streamlink/stream/dash/manifest.py#L859-L885
As you already figured out yourself, the suggested presentation delay has a static default value of 3 and it's missing an option for customizing it. I had a look at adding an option a few months ago, but didn't finish it because of some initialization issues I've run into which required more code refactoring of the MPD and DASHStream classes.
Respecting the manifest's min buffer time however sounds reasonable and should be fixed. | 2023-10-13T11:28:30 |
streamlink/streamlink | 5,616 | streamlink__streamlink-5616 | [
"5615"
] | c82a853567a4cb00fc4cc2007031b8865bff8bab | diff --git a/src/streamlink/plugins/dash.py b/src/streamlink/plugins/dash.py
--- a/src/streamlink/plugins/dash.py
+++ b/src/streamlink/plugins/dash.py
@@ -15,6 +15,7 @@
))
@pluginmatcher(priority=LOW_PRIORITY, pattern=re.compile(
r"(?P<url>\S+\.mpd(?:\?\S*)?)(?:\s(?P<params>.+))?$",
+ re.IGNORECASE,
))
class MPEGDASH(Plugin):
@classmethod
diff --git a/src/streamlink/plugins/hls.py b/src/streamlink/plugins/hls.py
--- a/src/streamlink/plugins/hls.py
+++ b/src/streamlink/plugins/hls.py
@@ -15,6 +15,7 @@
))
@pluginmatcher(priority=LOW_PRIORITY, pattern=re.compile(
r"(?P<url>\S+\.m3u8(?:\?\S*)?)(?:\s(?P<params>.+))?$",
+ re.IGNORECASE,
))
class HLSPlugin(Plugin):
def _get_streams(self):
| diff --git a/tests/plugins/test_dash.py b/tests/plugins/test_dash.py
--- a/tests/plugins/test_dash.py
+++ b/tests/plugins/test_dash.py
@@ -14,6 +14,7 @@ class TestPluginCanHandleUrlMPEGDASH(PluginCanHandleUrl):
should_match_groups = [
# implicit DASH URLs
("example.com/foo.mpd", {"url": "example.com/foo.mpd"}),
+ ("example.com/foo.MPD", {"url": "example.com/foo.MPD"}),
("example.com/foo.mpd?bar", {"url": "example.com/foo.mpd?bar"}),
("http://example.com/foo.mpd", {"url": "http://example.com/foo.mpd"}),
("http://example.com/foo.mpd?bar", {"url": "http://example.com/foo.mpd?bar"}),
@@ -33,6 +34,7 @@ class TestPluginCanHandleUrlMPEGDASH(PluginCanHandleUrl):
should_not_match = [
# implicit DASH URLs must have their path end with ".mpd"
"example.com/mpd",
+ "example.com/MPD",
"example.com/mpd abc=def",
"example.com/foo.mpd,bar",
"example.com/foo.mpd,bar abc=def",
@@ -44,6 +46,7 @@ class TestPluginCanHandleUrlMPEGDASH(PluginCanHandleUrl):
@pytest.mark.parametrize(("url", "priority"), [
("http://example.com/foo.mpd", LOW_PRIORITY),
+ ("http://example.com/foo.MPD", LOW_PRIORITY),
("dash://http://example.com/foo.mpd", NORMAL_PRIORITY),
("dash://http://example.com/bar", NORMAL_PRIORITY),
("http://example.com/bar", NO_PRIORITY),
diff --git a/tests/plugins/test_hls.py b/tests/plugins/test_hls.py
--- a/tests/plugins/test_hls.py
+++ b/tests/plugins/test_hls.py
@@ -15,6 +15,7 @@ class TestPluginCanHandleUrlHLSPlugin(PluginCanHandleUrl):
should_match_groups = [
# implicit HLS URLs
("example.com/foo.m3u8", {"url": "example.com/foo.m3u8"}),
+ ("example.com/foo.M3U8", {"url": "example.com/foo.M3U8"}),
("example.com/foo.m3u8?bar", {"url": "example.com/foo.m3u8?bar"}),
("http://example.com/foo.m3u8", {"url": "http://example.com/foo.m3u8"}),
("http://example.com/foo.m3u8?bar", {"url": "http://example.com/foo.m3u8?bar"}),
@@ -38,6 +39,7 @@ class TestPluginCanHandleUrlHLSPlugin(PluginCanHandleUrl):
should_not_match = [
# implicit HLS URLs must have their path end with ".m3u8"
"example.com/m3u8",
+ "example.com/M3U8",
"example.com/m3u8 abc=def",
"example.com/foo.m3u8,bar",
"example.com/foo.m3u8,bar abc=def",
@@ -50,6 +52,7 @@ class TestPluginCanHandleUrlHLSPlugin(PluginCanHandleUrl):
@pytest.mark.parametrize(("url", "priority"), [
("http://example.com/foo.m3u8", LOW_PRIORITY),
+ ("http://example.com/foo.M3U8", LOW_PRIORITY),
("hls://http://example.com/foo.m3u8", NORMAL_PRIORITY),
("hls://http://example.com/bar", NORMAL_PRIORITY),
("hlsvariant://http://example.com/foo.m3u8", NORMAL_PRIORITY),
| plugins.hls: recognize URLs with uppercase ".M3U8"
### Checklist
- [X] This is a bug report and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed bug reports](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22bug%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
streamlink 6.2.1+29.gc82a8535
### Description
Currently a URL with upper case M3U8, e.g. `https://example.com/live.M3U8` would not be recognized as an HLS URL.
### Debug log
```text
>streamlink https://example.com/live.M3U8 best --loglevel=debug
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.11.1
[cli][debug] OpenSSL: OpenSSL 1.1.1q 5 Jul 2022
[cli][debug] Streamlink: 6.2.1+29.gc82a8535
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.5.7
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.2
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.16.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.22.0
[cli][debug] trio-websocket: 0.9.2
[cli][debug] typing-extensions: 4.4.0
[cli][debug] urllib3: 1.26.15
[cli][debug] websocket-client: 1.5.1
[cli][debug] Arguments:
[cli][debug] url=https://example.com/live.M3U8
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --player=mpv.exe
[cli][debug] --stream-segment-threads=10
[cli][debug] --hls-segment-queue-threshold=0.0
error: No plugin can handle URL: https://example.com/live.M3U8
```
| 2023-10-15T16:41:34 |
|
streamlink/streamlink | 5,619 | streamlink__streamlink-5619 | [
"5618"
] | 25af0f3b35a68ec81b22beacbd12bdfb7e3ed242 | diff --git a/src/streamlink/stream/hls/m3u8.py b/src/streamlink/stream/hls/m3u8.py
--- a/src/streamlink/stream/hls/m3u8.py
+++ b/src/streamlink/stream/hls/m3u8.py
@@ -156,8 +156,11 @@ def __init__(self, base_uri: Optional[str] = None):
def create_stream_info(cls, streaminf: Mapping[str, Optional[str]], streaminfoclass=None):
program_id = streaminf.get("PROGRAM-ID")
- _bandwidth = streaminf.get("BANDWIDTH")
- bandwidth = 0 if not _bandwidth else round(int(_bandwidth), 1 - int(math.log10(int(_bandwidth))))
+ try:
+ bandwidth = int(streaminf.get("BANDWIDTH") or 0)
+ bandwidth = round(bandwidth, 1 - int(math.log10(bandwidth)))
+ except ValueError:
+ bandwidth = 0
_resolution = streaminf.get("RESOLUTION")
resolution = None if not _resolution else cls.parse_resolution(_resolution)
| diff --git a/tests/resources/hls/test_multivariant_bandwidth.m3u8 b/tests/resources/hls/test_multivariant_bandwidth.m3u8
new file mode 100644
--- /dev/null
+++ b/tests/resources/hls/test_multivariant_bandwidth.m3u8
@@ -0,0 +1,19 @@
+#EXTM3U
+#EXT-X-MEDIA:TYPE=VIDEO,GROUP-ID="chunked",NAME="1080p60",AUTOSELECT=NO,DEFAULT=NO
+#EXT-X-STREAM-INF:BANDWIDTH=0,CODECS="avc1.64002A,mp4a.40.2",RESOLUTION=1920x1080,VIDEO="chunked",FRAME-RATE=59.993
+chunked.m3u8
+#EXT-X-MEDIA:TYPE=VIDEO,GROUP-ID="audio_only",NAME="Audio Only",AUTOSELECT=NO,DEFAULT=NO
+#EXT-X-STREAM-INF:CODECS="mp4a.40.2",VIDEO="audio_only"
+audio_only.m3u8
+#EXT-X-MEDIA:TYPE=VIDEO,GROUP-ID="720p60",NAME="720p60",AUTOSELECT=YES,DEFAULT=YES
+#EXT-X-STREAM-INF:BANDWIDTH=3000000,CODECS="avc1.4D0020,mp4a.40.2",RESOLUTION=1280x720,VIDEO="720p60",FRAME-RATE=59.993
+720p60.m3u8
+#EXT-X-MEDIA:TYPE=VIDEO,GROUP-ID="480p30",NAME="480p",AUTOSELECT=YES,DEFAULT=YES
+#EXT-X-STREAM-INF:BANDWIDTH=1500000,CODECS="avc1.4D001F,mp4a.40.2",RESOLUTION=852x480,VIDEO="480p30",FRAME-RATE=29.996
+480p30.m3u8
+#EXT-X-MEDIA:TYPE=VIDEO,GROUP-ID="360p30",NAME="360p",AUTOSELECT=YES,DEFAULT=YES
+#EXT-X-STREAM-INF:BANDWIDTH=700000,CODECS="avc1.4D001E,mp4a.40.2",RESOLUTION=640x360,VIDEO="360p30",FRAME-RATE=29.996
+360p30.m3u8
+#EXT-X-MEDIA:TYPE=VIDEO,GROUP-ID="160p30",NAME="160p",AUTOSELECT=YES,DEFAULT=YES
+#EXT-X-STREAM-INF:BANDWIDTH=300000,CODECS="avc1.4D000C,mp4a.40.2",RESOLUTION=284x160,VIDEO="160p30",FRAME-RATE=29.996
+160p30.m3u8
diff --git a/tests/stream/hls/test_m3u8.py b/tests/stream/hls/test_m3u8.py
--- a/tests/stream/hls/test_m3u8.py
+++ b/tests/stream/hls/test_m3u8.py
@@ -4,9 +4,11 @@
import pytest
from streamlink.stream.hls import (
+ M3U8,
ByteRange,
DateRange,
ExtInf,
+ HLSPlaylist,
HLSSegment,
M3U8Parser,
Media,
@@ -551,3 +553,16 @@ def test_parse_date(self) -> None:
== [None, True, True, True, False, False, False, True, True, None]
assert [playlist.is_date_in_daterange(playlist.segments[3].date, daterange) for daterange in playlist.dateranges] \
== [None, True, True, True, False, False, False, False, False, None]
+
+ def test_parse_bandwidth(self) -> None:
+ with text("hls/test_multivariant_bandwidth.m3u8") as m3u8_fh:
+ playlist: M3U8[HLSSegment, HLSPlaylist] = parse_m3u8(m3u8_fh.read(), "http://mocked/", parser=M3U8Parser)
+
+ assert [(pl.stream_info.video, pl.stream_info.bandwidth) for pl in playlist.playlists] == [
+ ("chunked", 0),
+ ("audio_only", 0),
+ ("720p60", 3000000),
+ ("480p30", 1500000),
+ ("360p30", 700000),
+ ("160p30", 300000),
+ ]
| stream.hls: BANDWIDTH=0 raises ValueError: math domain error
### Checklist
- [X] This is a bug report and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed bug reports](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22bug%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
6.2.1
### Description
We have noticed that Streamlink does not work with Twitch Highlight videos. It fails to locate a 'playable stream.' VODs and clips, on the other hand, work without any issues.
Example Link: https://www.twitch.tv/videos/1946928063
### Debug log
```text
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.11.5
[cli][debug] OpenSSL: OpenSSL 3.0.9 30 May 2023
[cli][debug] Streamlink: 6.2.1
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.7.22
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.3
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.19.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.22.2
[cli][debug] trio-websocket: 0.11.1
[cli][debug] typing-extensions: 4.8.0
[cli][debug] urllib3: 2.0.6
[cli][debug] websocket-client: 1.6.3
[cli][debug] Arguments:
[cli][debug] url=https://www.twitch.tv/videos/1946928063
[cli][debug] --loglevel=debug
[cli][debug] --ffmpeg-ffmpeg=C:\Program Files\Streamlink\ffmpeg\ffmpeg.exe
[cli][info] Found matching plugin twitch for URL https://www.twitch.tv/videos/1946928063
[plugins.twitch][debug] Getting HLS streams for video ID 1946928063
[utils.l10n][debug] Language code: en_US
error: No playable streams found on this URL: https://www.twitch.tv/videos/1946928063
```
| 2023-10-16T14:34:31 |
|
streamlink/streamlink | 5,622 | streamlink__streamlink-5622 | [
"5611"
] | 26dc2f62989b65bb85dc7a71503783a988c767fa | diff --git a/src/streamlink/plugins/dlive.py b/src/streamlink/plugins/dlive.py
--- a/src/streamlink/plugins/dlive.py
+++ b/src/streamlink/plugins/dlive.py
@@ -86,7 +86,7 @@ def _get_streams_live(self, channel):
self.author = channel
self.title = livestream["title"]
- return HLSStream.parse_variant_playlist(self.session, self.URL_LIVE.format(username=username))
+ return HLSStream.parse_variant_playlist(self.session, self.URL_LIVE.format(username=username), headers={"Referer": "https://dlive.tv/"})
def _get_streams(self):
video = self.match.group("video")
| plugins.dlive: Failed to fetch segment | 403 Client Error
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
[cli][info] Your Streamlink version (6.2.1) is up to date!
### Description
I navigate to the folder where streamlink.exe is located and enter the command "streamlink.exe https://dlive.tv/cryptokaprika best". It doesn't matter which channel is specified, the same error comes up for all of them as of late.
Here is the complete output that is shown to me in the command line:
C:\Program Files\Streamlink\bin>streamlink.exe https://dlive.tv/cryptokaprika best
[cli][info] Found matching plugin dlive for URL https://dlive.tv/cryptokaprika
[cli][info] Available streams: src (worst, best)
[cli][info] Opening stream: src (hls)
[cli][info] Starting player: C:\Program Files\VideoLAN\VLC\vlc.exe
[stream.hls][error] Failed to fetch segment 79790: Unable to open URL: https://videos.prd.dlivecdn.com/dlive/0000079790.ts (403 Client Error: Forbidden for url: https://videos.prd.dlivecdn.com/dlive/0000079790.ts)
[stream.hls][error] Failed to fetch segment 79791: Unable to open URL: https://videos.prd.dlivecdn.com/dlive/0000079791.ts (403 Client Error: Forbidden for url: https://videos.prd.dlivecdn.com/dlive/0000079791.ts)
[cli][info] Stream ended
[cli][info] Closing currently open stream...
The VLC Media Player also starts, but I only get the following picture and am referred to the homepage: [https://imgur.com/a/NpuAHQ3](https://imgur.com/a/NpuAHQ3)
### Debug log
```text
C:\Program Files\Streamlink\bin>streamlink.exe --loglevel=debug https://dlive.tv/cryptokaprika best
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.11.5
[cli][debug] OpenSSL: OpenSSL 3.0.9 30 May 2023
[cli][debug] Streamlink: 6.2.1
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.7.22
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.3
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.19.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.22.2
[cli][debug] trio-websocket: 0.11.1
[cli][debug] typing-extensions: 4.8.0
[cli][debug] urllib3: 2.0.6
[cli][debug] websocket-client: 1.6.3
[cli][debug] Arguments:
[cli][debug] url=https://dlive.tv/cryptokaprika
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --ffmpeg-ffmpeg=C:\Program Files\Streamlink\ffmpeg\ffmpeg.exe
[cli][info] Found matching plugin dlive for URL https://dlive.tv/cryptokaprika
[plugins.dlive][debug] Getting live HLS streams for cryptokaprika
[utils.l10n][debug] Language code: en_US
[cli][info] Available streams: src (worst, best)
[cli][info] Opening stream: src (hls)
[cli][info] Starting player: C:\Program Files\VideoLAN\VLC\vlc.exe
[stream.hls][debug] Reloading playlist
[cli][debug] Pre-buffering 8192 bytes
[stream.hls][debug] First Sequence: 79786; Last Sequence: 79791
[stream.hls][debug] Start offset: 0; Duration: None; Start Sequence: 79786; End Sequence: 79791
[stream.hls][debug] Adding segment 79786 to queue
[stream.hls][debug] Adding segment 79787 to queue
[stream.hls][debug] Adding segment 79788 to queue
[stream.hls][debug] Adding segment 79789 to queue
[stream.hls][debug] Adding segment 79790 to queue
[stream.hls][debug] Adding segment 79791 to queue
[stream.segmented][debug] Closing worker thread
[stream.hls][debug] Writing segment 79786 to output
[stream.hls][debug] Segment 79786 complete
[cli.output][debug] Opening subprocess: ['C:\\Program Files\\VideoLAN\\VLC\\vlc.exe', '--input-title-format', 'https://dlive.tv/cryptokaprika', '-']
[stream.hls][debug] Writing segment 79787 to output
[stream.hls][debug] Segment 79787 complete
[stream.hls][debug] Writing segment 79788 to output
[stream.hls][debug] Segment 79788 complete
[stream.hls][debug] Writing segment 79789 to output
[stream.hls][debug] Segment 79789 complete
[cli][debug] Writing stream to output
[stream.hls][error] Failed to fetch segment 79790: Unable to open URL: https://videos.prd.dlivecdn.com/dlive/0000079790.ts (403 Client Error: Forbidden for url: https://videos.prd.dlivecdn.com/dlive/0000079790.ts)
[stream.hls][error] Failed to fetch segment 79791: Unable to open URL: https://videos.prd.dlivecdn.com/dlive/0000079791.ts (403 Client Error: Forbidden for url: https://videos.prd.dlivecdn.com/dlive/0000079791.ts)
[stream.segmented][debug] Closing writer thread
[cli][info] Stream ended
[cli][info] Closing currently open stream...
```
| 2023-10-18T05:37:59 |
||
streamlink/streamlink | 5,645 | streamlink__streamlink-5645 | [
"5575"
] | fd83550d5e4c55b84a2e96de70d0ea54f46eee10 | diff --git a/src/streamlink/plugins/tvp.py b/src/streamlink/plugins/tvp.py
--- a/src/streamlink/plugins/tvp.py
+++ b/src/streamlink/plugins/tvp.py
@@ -1,7 +1,9 @@
"""
$description Live TV channels and VODs from TVP, a Polish public, state-owned broadcaster.
+$url stream.tvp.pl
+$url vod.tvp.pl
+$url tvpstream.vod.tvp.pl
$url tvp.info
-$url tvp.pl
$type live, vod
$metadata id
$metadata title
@@ -23,21 +25,20 @@
log = logging.getLogger(__name__)
-@pluginmatcher(re.compile(r"""https?://(?:www\.)?tvp\.info/"""), name="tvp_info")
-@pluginmatcher(re.compile(
- r"""
- https?://
- (?:
- (?:tvpstream\.vod|stream)\.tvp\.pl(?:/(?:\?channel_id=(?P<channel_id>\d+))?)?$
- |
- vod\.tvp\.pl/[^/]+/.+,(?P<vod_id>\d+)$
- )
- """,
- re.VERBOSE,
+@pluginmatcher(name="default", pattern=re.compile(
+ r"https?://(?:stream|tvpstream\.vod)\.tvp\.pl(?:/(?:\?channel_id=(?P<channel_id>\d+))?)?$",
+))
+@pluginmatcher(name="vod", pattern=re.compile(
+ r"https?://vod\.tvp\.pl/[^/]+/.+,(?P<vod_id>\d+)$",
+))
+@pluginmatcher(name="tvp_info", pattern=re.compile(
+ r"https?://(?:www\.)?tvp\.info/",
))
class TVP(Plugin):
_URL_PLAYER = "https://stream.tvp.pl/sess/TVPlayer2/embed.php"
_URL_VOD = "https://vod.tvp.pl/api/products/{vod_id}/videos/playlist"
+ _URL_INFO_API_TOKEN = "https://api.tvp.pl/tokenizer/token/{token}"
+ _URL_INFO_API_NEWS = "https://www.tvp.info/api/info/news?device=www&id={id}"
def _get_video_id(self, channel_id: Optional[str]):
items: List[Tuple[int, int]] = self.session.http.get(
@@ -179,27 +180,44 @@ def _get_tvp_info_vod(self):
self.url,
schema=validate.Schema(
validate.parse_html(),
- validate.xml_xpath_string(".//script[contains(text(), 'window.__videoData')][1]/text()"),
+ validate.xml_xpath_string(".//script[contains(text(), 'window.__pageSettings')][1]/text()"),
validate.none_or_all(
str,
- validate.regex(re.compile(r"window\.__videoData\s*=\s*(?P<json>{.*?})\s*;", re.DOTALL)),
+ validate.regex(re.compile(r"window\.__pageSettings\s*=\s*(?P<json>{.*?})\s*;", re.DOTALL)),
validate.get("json"),
validate.parse_json(),
{
- "_id": int,
- "title": str,
+ "type": validate.any("VIDEO", "NEWS"),
+ "id": int,
},
+ validate.union_get("type", "id"),
),
),
)
if not data:
return
- self.id = data.get("_id")
- self.title = data.get("title")
+ _type, self.id = data
+ if _type == "NEWS":
+ self.id, self.title = self.session.http.get(
+ self._URL_INFO_API_NEWS.format(id=self.id),
+ schema=validate.Schema(
+ validate.parse_json(),
+ {
+ "data": {
+ "video": {
+ "title": str,
+ "_id": int,
+ },
+ },
+ },
+ validate.get(("data", "video")),
+ validate.union_get("_id", "title"),
+ ),
+ )
data = self.session.http.get(
- f"https://api.tvp.pl/tokenizer/token/{self.id}",
+ self._URL_INFO_API_TOKEN.format(token=self.id),
schema=validate.Schema(
validate.parse_json(),
{
@@ -225,10 +243,9 @@ def _get_tvp_info_vod(self):
def _get_streams(self):
if self.matches["tvp_info"]:
return self._get_tvp_info_vod()
- elif self.match["vod_id"]:
+ if self.matches["vod"]:
return self._get_vod(self.match["vod_id"])
- else:
- return self._get_live(self.match["channel_id"])
+ return self._get_live(self.match["channel_id"])
__plugin__ = TVP
| diff --git a/tests/plugins/test_tvp.py b/tests/plugins/test_tvp.py
--- a/tests/plugins/test_tvp.py
+++ b/tests/plugins/test_tvp.py
@@ -7,31 +7,30 @@ class TestPluginCanHandleUrlTVP(PluginCanHandleUrl):
should_match_groups = [
# live
- ("https://stream.tvp.pl", {}),
- ("https://stream.tvp.pl/", {}),
- ("https://stream.tvp.pl/?channel_id=63759349", {"channel_id": "63759349"}),
- ("https://stream.tvp.pl/?channel_id=14812849", {"channel_id": "14812849"}),
+ (("default", "https://stream.tvp.pl"), {}),
+ (("default", "https://stream.tvp.pl/"), {}),
+ (("default", "https://stream.tvp.pl/?channel_id=63759349"), {"channel_id": "63759349"}),
+ (("default", "https://stream.tvp.pl/?channel_id=14812849"), {"channel_id": "14812849"}),
# old live URLs
- ("https://tvpstream.vod.tvp.pl", {}),
- ("https://tvpstream.vod.tvp.pl/", {}),
- ("https://tvpstream.vod.tvp.pl/?channel_id=63759349", {"channel_id": "63759349"}),
- ("https://tvpstream.vod.tvp.pl/?channel_id=14812849", {"channel_id": "14812849"}),
+ (("default", "https://tvpstream.vod.tvp.pl"), {}),
+ (("default", "https://tvpstream.vod.tvp.pl/"), {}),
+ (("default", "https://tvpstream.vod.tvp.pl/?channel_id=63759349"), {"channel_id": "63759349"}),
+ (("default", "https://tvpstream.vod.tvp.pl/?channel_id=14812849"), {"channel_id": "14812849"}),
# VOD
(
- "https://vod.tvp.pl/filmy-dokumentalne,163/krolowa-wladczyni-i-matka,284734",
+ ("vod", "https://vod.tvp.pl/filmy-dokumentalne,163/krolowa-wladczyni-i-matka,284734"),
{"vod_id": "284734"},
),
# VOD episode
(
- "https://vod.tvp.pl/programy,88/z-davidem-attenborough-dokola-swiata-odcinki,284703/odcinek-2,S01E02,319220",
+ ("vod", "https://vod.tvp.pl/programy,88/z-davidem-attenborough-dokola-swiata-odcinki,284703/odcinek-2,S01E02,319220"),
{"vod_id": "319220"},
),
# tvp.info
- (("tvp_info", "https://tvp.info/"), {}),
- (("tvp_info", "https://www.tvp.info/"), {}),
- (("tvp_info", "https://www.tvp.info/65275202/13012023-0823"), {}),
+ (("tvp_info", "https://www.tvp.info/72577058/28092023-0823"), {}),
+ (("tvp_info", "https://www.tvp.info/73805503/przygotowania-do-uroczystosci-wszystkich-swietych"), {}),
]
should_not_match = [
| plugins.tvp: No playable streams found on www.tvp.info
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
6.2.0
### Description
It worked in the past but stopped some time ago:
streamlink https://www.tvp.info/72577058/28092023-0823 best --stream-url
error: No playable streams found on this URL: https://www.tvp.info/72577058/28092023-0823
### Debug log
```text
streamlink https://www.tvp.info/72577058/28092023-0823 best --stream-url --loglevel=debug
error: No playable streams found on this URL: https://www.tvp.info/72577058/28092023-0823
```
| 2023-10-31T17:42:38 |
|
streamlink/streamlink | 5,654 | streamlink__streamlink-5654 | [
"5582"
] | 003debed0ccb062f8de215b85886634283eac04e | diff --git a/src/streamlink/stream/dash/manifest.py b/src/streamlink/stream/dash/manifest.py
--- a/src/streamlink/stream/dash/manifest.py
+++ b/src/streamlink/stream/dash/manifest.py
@@ -632,7 +632,10 @@ def segments(self, timestamp: Optional[datetime] = None, **kwargs) -> Iterator[D
**kwargs,
)
elif segmentList:
- yield from segmentList.segments()
+ yield from segmentList.segments(
+ self.ident,
+ **kwargs,
+ )
else:
yield DASHSegment(
uri=self.base_url,
@@ -722,8 +725,13 @@ def __init__(self, *args, **kwargs) -> None:
self.segmentURLs = self.children(SegmentURL)
- def segments(self) -> Iterator[DASHSegment]:
- if self.initialization: # pragma: no branch
+ def segments(
+ self,
+ ident: TTimelineIdent,
+ **kwargs,
+ ) -> Iterator[DASHSegment]:
+ init = kwargs.pop("init", True)
+ if init and self.initialization: # pragma: no branch
yield DASHSegment(
uri=self.make_url(self.initialization.source_url),
num=-1,
@@ -733,9 +741,23 @@ def segments(self) -> Iterator[DASHSegment]:
content=False,
byterange=self.initialization.range,
)
+
+ if init:
+ # yield all segments initially and remember the segment number
+ start_number = self.startNumber
+ segment_urls = self.segmentURLs
+ self.root.timelines[ident] = start_number
+ else:
+ # skip segments with a lower number than the remembered segment number
+ start_number = self.root.timelines[ident]
+ segment_urls = self.segmentURLs[start_number - self.startNumber:]
+
+ # add the number of yielded segments to the remembered segment number
+ self.root.timelines[ident] += len(segment_urls)
+
num: int
segment_url: SegmentURL
- for num, segment_url in enumerate(self.segmentURLs, self.startNumber):
+ for num, segment_url in enumerate(segment_urls, start_number):
yield DASHSegment(
uri=self.make_url(segment_url.media),
num=num,
| diff --git a/tests/resources/dash/test_dynamic_segment_list_p1.mpd b/tests/resources/dash/test_dynamic_segment_list_p1.mpd
new file mode 100644
--- /dev/null
+++ b/tests/resources/dash/test_dynamic_segment_list_p1.mpd
@@ -0,0 +1,33 @@
+<?xml version="1.0" encoding="UTF-8"?>
+<MPD
+ xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
+ xmlns="urn:mpeg:dash:schema:mpd:2011"
+ xsi:schemaLocation="urn:mpeg:dash:schema:mpd:2011 DASH-MPD.xsd"
+ profiles="urn:mpeg:dash:profile:isoff-live:2011,http://dashif.org/guidelines/dash-if-simple"
+ type="dynamic"
+ availabilityStartTime="1970-01-01T00:00:00Z"
+ publishTime="2000-01-01T00:00:00Z"
+ maxSegmentDuration="PT10S"
+ minBufferTime="PT10S"
+>
+<Period id="0" start="PT0S">
+ <AdaptationSet id="0" group="1" mimeType="video/mp4" maxWidth="1920" maxHeight="1080" par="16:9" frameRate="25" segmentAlignment="true" startWithSAP="1" subsegmentAlignment="true" subsegmentStartsWithSAP="1">
+ <Representation id="0" codecs="avc1.640028" width="1920" height="1080" sar="1:1" bandwidth="4332748">
+ <SegmentList presentationTimeOffset="0" timescale="90000" duration="900000" startNumber="5">
+ <Initialization sourceURL="init.m4s"/>
+ <SegmentURL media="5.m4s"/>
+ <SegmentURL media="6.m4s"/>
+ <SegmentURL media="7.m4s"/>
+ <SegmentURL media="8.m4s"/>
+ <SegmentURL media="9.m4s"/>
+ <SegmentURL media="10.m4s"/>
+ <SegmentURL media="11.m4s"/>
+ <SegmentURL media="12.m4s"/>
+ <SegmentURL media="13.m4s"/>
+ <SegmentURL media="14.m4s"/>
+ <SegmentURL media="15.m4s"/>
+ </SegmentList>
+ </Representation>
+ </AdaptationSet>
+</Period>
+</MPD>
diff --git a/tests/resources/dash/test_dynamic_segment_list_p2.mpd b/tests/resources/dash/test_dynamic_segment_list_p2.mpd
new file mode 100644
--- /dev/null
+++ b/tests/resources/dash/test_dynamic_segment_list_p2.mpd
@@ -0,0 +1,32 @@
+<?xml version="1.0" encoding="UTF-8"?>
+<MPD
+ xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
+ xmlns="urn:mpeg:dash:schema:mpd:2011"
+ xsi:schemaLocation="urn:mpeg:dash:schema:mpd:2011 DASH-MPD.xsd"
+ profiles="urn:mpeg:dash:profile:isoff-live:2011,http://dashif.org/guidelines/dash-if-simple"
+ type="dynamic"
+ availabilityStartTime="1970-01-01T00:00:00Z"
+ publishTime="2000-01-01T00:00:00Z"
+ maxSegmentDuration="PT10S"
+ minBufferTime="PT10S"
+>
+<Period id="0" start="PT0S">
+ <AdaptationSet id="0" group="1" mimeType="video/mp4" maxWidth="1920" maxHeight="1080" par="16:9" frameRate="25" segmentAlignment="true" startWithSAP="1" subsegmentAlignment="true" subsegmentStartsWithSAP="1">
+ <Representation id="0" codecs="avc1.640028" width="1920" height="1080" sar="1:1" bandwidth="4332748">
+ <SegmentList presentationTimeOffset="0" timescale="90000" duration="900000" startNumber="9">
+ <Initialization sourceURL="init.m4s"/>
+ <SegmentURL media="9.m4s"/>
+ <SegmentURL media="10.m4s"/>
+ <SegmentURL media="11.m4s"/>
+ <SegmentURL media="12.m4s"/>
+ <SegmentURL media="13.m4s"/>
+ <SegmentURL media="14.m4s"/>
+ <SegmentURL media="15.m4s"/>
+ <SegmentURL media="16.m4s"/>
+ <SegmentURL media="17.m4s"/>
+ <SegmentURL media="18.m4s"/>
+ </SegmentList>
+ </Representation>
+ </AdaptationSet>
+</Period>
+</MPD>
diff --git a/tests/resources/dash/test_dynamic_segment_list_p3.mpd b/tests/resources/dash/test_dynamic_segment_list_p3.mpd
new file mode 100644
--- /dev/null
+++ b/tests/resources/dash/test_dynamic_segment_list_p3.mpd
@@ -0,0 +1,32 @@
+<?xml version="1.0" encoding="UTF-8"?>
+<MPD
+ xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
+ xmlns="urn:mpeg:dash:schema:mpd:2011"
+ xsi:schemaLocation="urn:mpeg:dash:schema:mpd:2011 DASH-MPD.xsd"
+ profiles="urn:mpeg:dash:profile:isoff-live:2011,http://dashif.org/guidelines/dash-if-simple"
+ type="dynamic"
+ availabilityStartTime="1970-01-01T00:00:00Z"
+ publishTime="2000-01-01T00:00:00Z"
+ maxSegmentDuration="PT10S"
+ minBufferTime="PT10S"
+>
+<Period id="0" start="PT0S">
+ <AdaptationSet id="0" group="1" mimeType="video/mp4" maxWidth="1920" maxHeight="1080" par="16:9" frameRate="25" segmentAlignment="true" startWithSAP="1" subsegmentAlignment="true" subsegmentStartsWithSAP="1">
+ <Representation id="0" codecs="avc1.640028" width="1920" height="1080" sar="1:1" bandwidth="4332748">
+ <SegmentList presentationTimeOffset="0" timescale="90000" duration="900000" startNumber="12">
+ <Initialization sourceURL="init.m4s"/>
+ <SegmentURL media="12.m4s"/>
+ <SegmentURL media="13.m4s"/>
+ <SegmentURL media="14.m4s"/>
+ <SegmentURL media="15.m4s"/>
+ <SegmentURL media="16.m4s"/>
+ <SegmentURL media="17.m4s"/>
+ <SegmentURL media="18.m4s"/>
+ <SegmentURL media="19.m4s"/>
+ <SegmentURL media="20.m4s"/>
+ <SegmentURL media="21.m4s"/>
+ </SegmentList>
+ </Representation>
+ </AdaptationSet>
+</Period>
+</MPD>
diff --git a/tests/stream/dash/test_manifest.py b/tests/stream/dash/test_manifest.py
--- a/tests/stream/dash/test_manifest.py
+++ b/tests/stream/dash/test_manifest.py
@@ -276,6 +276,46 @@ def test_segment_list(self):
("http://test/chunk_ctvideo_ridp0va0br4332748_cn3_mpd.m4s", expected_availability),
]
+ def test_dynamic_segment_list_continued(self):
+ with xml("dash/test_dynamic_segment_list_p1.mpd") as mpd_xml:
+ mpd = MPD(mpd_xml, base_url="http://test/", url="http://test/manifest.mpd")
+
+ segments_iterator = mpd.periods[0].adaptationSets[0].representations[0].segments(init=True)
+ assert [segment.uri for segment in segments_iterator] == [
+ "http://test/init.m4s",
+ "http://test/5.m4s",
+ "http://test/6.m4s",
+ "http://test/7.m4s",
+ "http://test/8.m4s",
+ "http://test/9.m4s",
+ "http://test/10.m4s",
+ "http://test/11.m4s",
+ "http://test/12.m4s",
+ "http://test/13.m4s",
+ "http://test/14.m4s",
+ "http://test/15.m4s",
+ ]
+
+ with xml("dash/test_dynamic_segment_list_p2.mpd") as mpd_xml:
+ mpd = MPD(mpd_xml, base_url="http://test/", url="http://test/manifest.mpd", timelines=mpd.timelines)
+
+ segments_iterator = mpd.periods[0].adaptationSets[0].representations[0].segments(init=False)
+ assert [segment.uri for segment in segments_iterator] == [
+ "http://test/16.m4s",
+ "http://test/17.m4s",
+ "http://test/18.m4s",
+ ]
+
+ with xml("dash/test_dynamic_segment_list_p3.mpd") as mpd_xml:
+ mpd = MPD(mpd_xml, base_url="http://test/", url="http://test/manifest.mpd", timelines=mpd.timelines)
+
+ segments_iterator = mpd.periods[0].adaptationSets[0].representations[0].segments(init=False)
+ assert [segment.uri for segment in segments_iterator] == [
+ "http://test/19.m4s",
+ "http://test/20.m4s",
+ "http://test/21.m4s",
+ ]
+
def test_dynamic_timeline_continued(self):
with xml("dash/test_dynamic_timeline_continued_p1.mpd") as mpd_xml_p1:
mpd_p1 = MPD(mpd_xml_p1, base_url="http://test/", url="http://test/manifest.mpd")
| stream.dash: support for dynamic manifests with SegmentLists
### Checklist
- [X] This is a bug report and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed bug reports](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22bug%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
6.2.1
### Description
TLDR: It seems that the dash implementation doesn't filter the segments when a reload has happened, causing all segments to be downloaded again.
I have a livestream with a manifest that contains below SegmentList:
```
...
<Representation id="video-5" mimeType="video/mp4" codecs="avc1.64001e" bandwidth="4600000" width="1920" height="1080">
<SegmentList presentationTimeOffset="52459080" timescale="1000" duration="4000" startNumber="2">
<Initialization sourceURL="4792k/ugSxF45MOHE/init-video.mp4?hdntl=exp=1696435540~acl=/*~hmac=1eba0a04cd7d2ec0d55a3a1e2317a9d77d375b1f8a42f0926914bf7575aebd61" />
<SegmentURL media="4792k/ugSxF45MOHE/178185/segment_178185772-video.mp4?hdntl=exp=1696435540~acl=/*~hmac=1eba0a04cd7d2ec0d55a3a1e2317a9d77d375b1f8a42f0926914bf7575aebd61"/>
<SegmentURL media="4792k/ugSxF45MOHE/178185/segment_178185773-video.mp4?hdntl=exp=1696435540~acl=/*~hmac=1eba0a04cd7d2ec0d55a3a1e2317a9d77d375b1f8a42f0926914bf7575aebd61"/>
<SegmentURL media="4792k/ugSxF45MOHE/178185/segment_178185774-video.mp4?hdntl=exp=1696435540~acl=/*~hmac=1eba0a04cd7d2ec0d55a3a1e2317a9d77d375b1f8a42f0926914bf7575aebd61"/>
<SegmentURL media="4792k/ugSxF45MOHE/178185/segment_178185775-video.mp4?hdntl=exp=1696435540~acl=/*~hmac=1eba0a04cd7d2ec0d55a3a1e2317a9d77d375b1f8a42f0926914bf7575aebd61"/>
<SegmentURL media="4792k/ugSxF45MOHE/178185/segment_178185776-video.mp4?hdntl=exp=1696435540~acl=/*~hmac=1eba0a04cd7d2ec0d55a3a1e2317a9d77d375b1f8a42f0926914bf7575aebd61"/>
<SegmentURL media="4792k/ugSxF45MOHE/178185/segment_178185777-video.mp4?hdntl=exp=1696435540~acl=/*~hmac=1eba0a04cd7d2ec0d55a3a1e2317a9d77d375b1f8a42f0926914bf7575aebd61"/>
<SegmentURL media="4792k/ugSxF45MOHE/178185/segment_178185778-video.mp4?hdntl=exp=1696435540~acl=/*~hmac=1eba0a04cd7d2ec0d55a3a1e2317a9d77d375b1f8a42f0926914bf7575aebd61"/>
<SegmentURL media="4792k/ugSxF45MOHE/178185/segment_178185779-video.mp4?hdntl=exp=1696435540~acl=/*~hmac=1eba0a04cd7d2ec0d55a3a1e2317a9d77d375b1f8a42f0926914bf7575aebd61"/>
<SegmentURL media="4792k/ugSxF45MOHE/178185/segment_178185780-video.mp4?hdntl=exp=1696435540~acl=/*~hmac=1eba0a04cd7d2ec0d55a3a1e2317a9d77d375b1f8a42f0926914bf7575aebd61"/>
<SegmentURL media="4792k/ugSxF45MOHE/178185/segment_178185781-video.mp4?hdntl=exp=1696435540~acl=/*~hmac=1eba0a04cd7d2ec0d55a3a1e2317a9d77d375b1f8a42f0926914bf7575aebd61"/>
<SegmentURL media="4792k/ugSxF45MOHE/178185/segment_178185782-video.mp4?hdntl=exp=1696435540~acl=/*~hmac=1eba0a04cd7d2ec0d55a3a1e2317a9d77d375b1f8a42f0926914bf7575aebd61"/>
<SegmentURL media="4792k/ugSxF45MOHE/178185/segment_178185783-video.mp4?hdntl=exp=1696435540~acl=/*~hmac=1eba0a04cd7d2ec0d55a3a1e2317a9d77d375b1f8a42f0926914bf7575aebd61"/>
<SegmentURL media="4792k/ugSxF45MOHE/178185/segment_178185784-video.mp4?hdntl=exp=1696435540~acl=/*~hmac=1eba0a04cd7d2ec0d55a3a1e2317a9d77d375b1f8a42f0926914bf7575aebd61"/>
<SegmentURL media="4792k/ugSxF45MOHE/178185/segment_178185785-video.mp4?hdntl=exp=1696435540~acl=/*~hmac=1eba0a04cd7d2ec0d55a3a1e2317a9d77d375b1f8a42f0926914bf7575aebd61"/>
<SegmentURL media="4792k/ugSxF45MOHE/178185/segment_178185786-video.mp4?hdntl=exp=1696435540~acl=/*~hmac=1eba0a04cd7d2ec0d55a3a1e2317a9d77d375b1f8a42f0926914bf7575aebd61"/>
<SegmentURL media="4792k/ugSxF45MOHE/178185/segment_178185787-video.mp4?hdntl=exp=1696435540~acl=/*~hmac=1eba0a04cd7d2ec0d55a3a1e2317a9d77d375b1f8a42f0926914bf7575aebd61"/>
</SegmentList>
</Representation>
...
```
Nothing really spectacular here, but it seems that the DASH code starts downloading from the top, instead of only the last 3 as with HLS. This is probably a different feature request, but it's somewhat related.
The issue is that after a reload, the whole manifest is reloaded, and it starts downloading at the top again, instead of only the new Segments.
After reload:
```
...
<Representation id="video-5" mimeType="video/mp4" codecs="avc1.64001e" bandwidth="4600000" width="1920" height="1080">
<SegmentList presentationTimeOffset="52459080" timescale="1000" duration="4000" startNumber="4">
<Initialization sourceURL="4792k/ugSxF45MOHE/init-video.mp4?hdntl=exp=1696435545~acl=/*~hmac=a0a36aa15b7c308b9a9e764777805c4ecfb09be9f410767484a1715c25ddfc39" />
<SegmentURL media="4792k/ugSxF45MOHE/178185/segment_178185774-video.mp4?hdntl=exp=1696435545~acl=/*~hmac=a0a36aa15b7c308b9a9e764777805c4ecfb09be9f410767484a1715c25ddfc39"/>
<SegmentURL media="4792k/ugSxF45MOHE/178185/segment_178185775-video.mp4?hdntl=exp=1696435545~acl=/*~hmac=a0a36aa15b7c308b9a9e764777805c4ecfb09be9f410767484a1715c25ddfc39"/>
<SegmentURL media="4792k/ugSxF45MOHE/178185/segment_178185776-video.mp4?hdntl=exp=1696435545~acl=/*~hmac=a0a36aa15b7c308b9a9e764777805c4ecfb09be9f410767484a1715c25ddfc39"/>
<SegmentURL media="4792k/ugSxF45MOHE/178185/segment_178185777-video.mp4?hdntl=exp=1696435545~acl=/*~hmac=a0a36aa15b7c308b9a9e764777805c4ecfb09be9f410767484a1715c25ddfc39"/>
<SegmentURL media="4792k/ugSxF45MOHE/178185/segment_178185778-video.mp4?hdntl=exp=1696435545~acl=/*~hmac=a0a36aa15b7c308b9a9e764777805c4ecfb09be9f410767484a1715c25ddfc39"/>
<SegmentURL media="4792k/ugSxF45MOHE/178185/segment_178185779-video.mp4?hdntl=exp=1696435545~acl=/*~hmac=a0a36aa15b7c308b9a9e764777805c4ecfb09be9f410767484a1715c25ddfc39"/>
<SegmentURL media="4792k/ugSxF45MOHE/178185/segment_178185780-video.mp4?hdntl=exp=1696435545~acl=/*~hmac=a0a36aa15b7c308b9a9e764777805c4ecfb09be9f410767484a1715c25ddfc39"/>
<SegmentURL media="4792k/ugSxF45MOHE/178185/segment_178185781-video.mp4?hdntl=exp=1696435545~acl=/*~hmac=a0a36aa15b7c308b9a9e764777805c4ecfb09be9f410767484a1715c25ddfc39"/>
<SegmentURL media="4792k/ugSxF45MOHE/178185/segment_178185782-video.mp4?hdntl=exp=1696435545~acl=/*~hmac=a0a36aa15b7c308b9a9e764777805c4ecfb09be9f410767484a1715c25ddfc39"/>
<SegmentURL media="4792k/ugSxF45MOHE/178185/segment_178185783-video.mp4?hdntl=exp=1696435545~acl=/*~hmac=a0a36aa15b7c308b9a9e764777805c4ecfb09be9f410767484a1715c25ddfc39"/>
<SegmentURL media="4792k/ugSxF45MOHE/178185/segment_178185784-video.mp4?hdntl=exp=1696435545~acl=/*~hmac=a0a36aa15b7c308b9a9e764777805c4ecfb09be9f410767484a1715c25ddfc39"/>
<SegmentURL media="4792k/ugSxF45MOHE/178185/segment_178185785-video.mp4?hdntl=exp=1696435545~acl=/*~hmac=a0a36aa15b7c308b9a9e764777805c4ecfb09be9f410767484a1715c25ddfc39"/>
<SegmentURL media="4792k/ugSxF45MOHE/178185/segment_178185786-video.mp4?hdntl=exp=1696435545~acl=/*~hmac=a0a36aa15b7c308b9a9e764777805c4ecfb09be9f410767484a1715c25ddfc39"/>
<SegmentURL media="4792k/ugSxF45MOHE/178185/segment_178185787-video.mp4?hdntl=exp=1696435545~acl=/*~hmac=a0a36aa15b7c308b9a9e764777805c4ecfb09be9f410767484a1715c25ddfc39"/>
<SegmentURL media="4792k/ugSxF45MOHE/178185/segment_178185788-video.mp4?hdntl=exp=1696435545~acl=/*~hmac=a0a36aa15b7c308b9a9e764777805c4ecfb09be9f410767484a1715c25ddfc39"/>
<SegmentURL media="4792k/ugSxF45MOHE/178185/segment_178185789-video.mp4?hdntl=exp=1696435545~acl=/*~hmac=a0a36aa15b7c308b9a9e764777805c4ecfb09be9f410767484a1715c25ddfc39"/>
</SegmentList>
</Representation>
...
```
I've looked at the code, and it seems the whole playlist is reloaded in `stream.dash.reload()` and there is no code to filter out already downloaded segments.
I'm using streamlink through the Python API, with `.open()` and `.read()`, hence the timestamps and thread names in the debug output.
### Debug log
```text
2023-10-04 10:17:31,764.764 debug MainThread streamlink.plugins.dash URL=https://dcs-live-uw1.mp.lura.live/server/play/OV3pDTvQYW5cMWeXW/manifest.mpd?anvsid=m177610521-na549ea847f984f7defa73e3830473903; params={}
2023-10-04 10:17:31,766.766 debug MainThread urllib3.connectionpool Starting new HTTPS connection (1): dcs-live-uw1.mp.lura.live:443
2023-10-04 10:17:32,014.014 debug MainThread urllib3.connectionpool https://dcs-live-uw1.mp.lura.live:443 "GET /server/play/OV3pDTvQYW5cMWeXW/manifest.mpd?anvsid=m177610521-na549ea847f984f7defa73e3830473903 HTTP/1.1" 200 None
2023-10-04 10:17:32,016.016 debug MainThread charset_normalizer Encoding detection: utf_8 is most likely the one.
2023-10-04 10:17:32,026.026 debug MainThread streamlink.utils.l10n Language code: en_US
2023-10-04 10:17:32,026.026 debug MainThread streamlink.stream.dash Available languages for DASH audio streams: NONE (using: n/a)
2023-10-04 10:17:32,027.027 info MainThread iptvstreamer Opening stream to https://dcs-live-uw1.mp.lura.live/server/play/OV3pDTvQYW5cMWeXW/manifest.mpd?anvsid=m177610521-na549ea847f984f7defa73e3830473903
2023-10-04 10:17:32,029.029 debug MainThread streamlink.stream.dash Opening DASH reader for: ('0', None, 'video-5') - video/mp4
2023-10-04 10:17:32,034.034 debug ThreadPoolExecutor-0_0 streamlink.stream.dash video/mp4 segment initialization: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:32.034361Z)
2023-10-04 10:17:32,036.036 debug MainThread streamlink.stream.dash Opening DASH reader for: ('0', None, 'audio-5') - audio/mp4
2023-10-04 10:17:32,038.038 debug ThreadPoolExecutor-0_0 urllib3.connectionpool Starting new HTTPS connection (1): video-live.dpgmedia.net:443
2023-10-04 10:17:32,040.040 debug Thread-DASHStreamWorker streamlink.stream.dash Reloading manifest ('0', None, 'video-5')
2023-10-04 10:17:32,046.046 debug MainThread asyncio Using selector: EpollSelector
2023-10-04 10:17:32,046.046 debug ThreadPoolExecutor-1_0 streamlink.stream.dash audio/mp4 segment initialization: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:32.046390Z)
2023-10-04 10:17:32,048.048 debug Thread-DASHStreamWorker streamlink.stream.dash Reloading manifest ('0', None, 'audio-5')
2023-10-04 10:17:32,054.054 debug ThreadPoolExecutor-1_0 urllib3.connectionpool Starting new HTTPS connection (2): video-live.dpgmedia.net:443
2023-10-04 10:17:32,055.055 debug Thread-DASHStreamWorker urllib3.connectionpool Starting new HTTPS connection (2): dcs-live-uw1.mp.lura.live:443
2023-10-04 10:17:32,060.060 debug MainThread streamlink.stream.ffmpegmux ffmpeg version 6.0-static https://johnvansickle.com/ffmpeg/ Copyright (c) 2000-2023 the FFmpeg developers
2023-10-04 10:17:32,060.060 debug MainThread streamlink.stream.ffmpegmux built with gcc 8 (Debian 8.3.0-6)
2023-10-04 10:17:32,060.060 debug MainThread streamlink.stream.ffmpegmux configuration: --enable-gpl --enable-version3 --enable-static --disable-debug --disable-ffplay --disable-indev=sndio --disable-outdev=sndio --cc=gcc --enable-fontconfig --enable-frei0r --enable-gnutls --enable-gmp --enable-libgme --enable-gray --enable-libaom --enable-libfribidi --enable-libass --enable-libvmaf --enable-libfreetype --enable-libmp3lame --enable-libopencore-amrnb --enable-libopencore-amrwb --enable-libopenjpeg --enable-librubberband --enable-libsoxr --enable-libspeex --enable-libsrt --enable-libvorbis --enable-libopus --enable-libtheora --enable-libvidstab --enable-libvo-amrwbenc --enable-libvpx --enable-libwebp --enable-libx264 --enable-libx265 --enable-libxml2 --enable-libdav1d --enable-libxvid --enable-libzvbi --enable-libzimg
2023-10-04 10:17:32,060.060 debug MainThread streamlink.stream.ffmpegmux libavutil 58. 2.100 / 58. 2.100
2023-10-04 10:17:32,060.060 debug MainThread streamlink.stream.ffmpegmux libavcodec 60. 3.100 / 60. 3.100
2023-10-04 10:17:32,060.060 debug MainThread streamlink.stream.ffmpegmux libavformat 60. 3.100 / 60. 3.100
2023-10-04 10:17:32,061.061 debug MainThread streamlink.stream.ffmpegmux libavdevice 60. 1.100 / 60. 1.100
2023-10-04 10:17:32,061.061 debug MainThread streamlink.stream.ffmpegmux libavfilter 9. 3.100 / 9. 3.100
2023-10-04 10:17:32,061.061 debug MainThread streamlink.stream.ffmpegmux libswscale 7. 1.100 / 7. 1.100
2023-10-04 10:17:32,061.061 debug MainThread streamlink.stream.ffmpegmux libswresample 4. 10.100 / 4. 10.100
2023-10-04 10:17:32,061.061 debug MainThread streamlink.stream.ffmpegmux libpostproc 57. 1.100 / 57. 1.100
2023-10-04 10:17:32,061.061 info MainThread streamlink.utils.named_pipe Creating pipe streamlinkpipe-9-1-4469
2023-10-04 10:17:32,062.062 info MainThread streamlink.utils.named_pipe Creating pipe streamlinkpipe-9-2-6040
2023-10-04 10:17:32,062.062 debug MainThread streamlink.stream.ffmpegmux ffmpeg command: /usr/local/bin/ffmpeg -nostats -y -i /tmp/streamlinkpipe-9-1-4469 -i /tmp/streamlinkpipe-9-2-6040 -c:v copy -c:a copy -copyts -start_at_zero -f mpegts pipe:1
2023-10-04 10:17:32,063.063 debug Thread-1 (copy_to_pipe) streamlink.stream.ffmpegmux Starting copy to pipe: /tmp/streamlinkpipe-9-1-4469
2023-10-04 10:17:32,063.063 debug Thread-2 (copy_to_pipe) streamlink.stream.ffmpegmux Starting copy to pipe: /tmp/streamlinkpipe-9-2-6040
2023-10-04 10:17:32,163.163 debug ThreadPoolExecutor-0_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/init-video.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 1280
2023-10-04 10:17:32,163.163 debug ThreadPoolExecutor-0_0 streamlink.stream.dash video/mp4 segment 1: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:32.163452Z)
2023-10-04 10:17:32,164.164 debug Thread-DASHStreamWriter streamlink.stream.dash video/mp4 segment initialization: completed
2023-10-04 10:17:32,186.186 debug ThreadPoolExecutor-0_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185950-video.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 1745420
2023-10-04 10:17:32,192.192 debug ThreadPoolExecutor-1_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/init-audio.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 1208
2023-10-04 10:17:32,192.192 debug ThreadPoolExecutor-1_0 streamlink.stream.dash audio/mp4 segment 1: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:32.192871Z)
2023-10-04 10:17:32,193.193 debug Thread-DASHStreamWriter streamlink.stream.dash audio/mp4 segment initialization: completed
2023-10-04 10:17:32,214.214 debug Thread-DASHStreamWorker urllib3.connectionpool https://dcs-live-uw1.mp.lura.live:443 "GET /server/play/OV3pDTvQYW5cMWeXW/manifest.mpd?anvsid=m177610521-na549ea847f984f7defa73e3830473903 HTTP/1.1" 200 None
2023-10-04 10:17:32,216.216 debug Thread-DASHStreamWorker charset_normalizer Encoding detection: utf_8 is most likely the one.
2023-10-04 10:17:32,221.221 debug ThreadPoolExecutor-1_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185950-audio.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 103963
2023-10-04 10:17:32,350.350 debug Thread-DASHStreamWorker urllib3.connectionpool https://dcs-live-uw1.mp.lura.live:443 "GET /server/play/OV3pDTvQYW5cMWeXW/manifest.mpd?anvsid=m177610521-na549ea847f984f7defa73e3830473903 HTTP/1.1" 200 None
2023-10-04 10:17:32,371.371 debug Thread-DASHStreamWorker charset_normalizer Encoding detection: utf_8 is most likely the one.
2023-10-04 10:17:32,379.379 debug ThreadPoolExecutor-0_0 streamlink.stream.dash video/mp4 segment 2: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:32.379296Z)
2023-10-04 10:17:32,382.382 debug Thread-DASHStreamWriter streamlink.stream.dash video/mp4 segment 1: completed
2023-10-04 10:17:32,403.403 debug ThreadPoolExecutor-0_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185951-video.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 1880960
2023-10-04 10:17:32,529.529 debug ThreadPoolExecutor-1_0 streamlink.stream.dash audio/mp4 segment 2: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:32.529373Z)
2023-10-04 10:17:32,531.531 debug Thread-DASHStreamWriter streamlink.stream.dash audio/mp4 segment 1: completed
2023-10-04 10:17:32,572.572 debug ThreadPoolExecutor-1_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185951-audio.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 100474
2023-10-04 10:17:32,589.589 debug ThreadPoolExecutor-1_0 streamlink.stream.dash audio/mp4 segment 3: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:32.589479Z)
2023-10-04 10:17:32,591.591 debug Thread-DASHStreamWriter streamlink.stream.dash audio/mp4 segment 2: completed
2023-10-04 10:17:32,592.592 debug ThreadPoolExecutor-0_0 streamlink.stream.dash video/mp4 segment 3: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:32.592798Z)
2023-10-04 10:17:32,594.594 debug Thread-DASHStreamWriter streamlink.stream.dash video/mp4 segment 2: completed
2023-10-04 10:17:32,609.609 debug ThreadPoolExecutor-1_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185952-audio.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 102117
2023-10-04 10:17:32,616.616 debug ThreadPoolExecutor-0_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185952-video.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 1749488
2023-10-04 10:17:32,664.664 debug ThreadPoolExecutor-1_0 streamlink.stream.dash audio/mp4 segment 4: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:32.664882Z)
2023-10-04 10:17:32,665.665 debug Thread-DASHStreamWriter streamlink.stream.dash audio/mp4 segment 3: completed
2023-10-04 10:17:32,690.690 debug ThreadPoolExecutor-1_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185953-audio.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 99864
2023-10-04 10:17:32,743.743 debug ThreadPoolExecutor-1_0 streamlink.stream.dash audio/mp4 segment 5: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:32.743061Z)
2023-10-04 10:17:32,743.743 debug Thread-DASHStreamWriter streamlink.stream.dash audio/mp4 segment 4: completed
2023-10-04 10:17:32,789.789 debug ThreadPoolExecutor-1_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185954-audio.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 104877
2023-10-04 10:17:32,798.798 debug ThreadPoolExecutor-1_0 streamlink.stream.dash audio/mp4 segment 6: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:32.798310Z)
2023-10-04 10:17:32,799.799 debug Thread-DASHStreamWriter streamlink.stream.dash audio/mp4 segment 5: completed
2023-10-04 10:17:32,803.803 debug ThreadPoolExecutor-0_0 streamlink.stream.dash video/mp4 segment 4: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:32.803131Z)
2023-10-04 10:17:32,805.805 debug Thread-DASHStreamWriter streamlink.stream.dash video/mp4 segment 3: completed
2023-10-04 10:17:32,819.819 debug ThreadPoolExecutor-1_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185955-audio.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 100514
2023-10-04 10:17:32,827.827 debug ThreadPoolExecutor-0_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185953-video.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 1762684
2023-10-04 10:17:32,832.832 debug ThreadPoolExecutor-1_0 streamlink.stream.dash audio/mp4 segment 7: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:32.832448Z)
2023-10-04 10:17:32,832.832 debug Thread-DASHStreamWriter streamlink.stream.dash audio/mp4 segment 6: completed
2023-10-04 10:17:32,903.903 debug ThreadPoolExecutor-1_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185956-audio.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 102101
2023-10-04 10:17:32,909.909 debug ThreadPoolExecutor-1_0 streamlink.stream.dash audio/mp4 segment 8: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:32.909266Z)
2023-10-04 10:17:32,909.909 debug Thread-DASHStreamWriter streamlink.stream.dash audio/mp4 segment 7: completed
2023-10-04 10:17:32,937.937 debug ThreadPoolExecutor-1_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185957-audio.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 103303
2023-10-04 10:17:33,001.001 debug ThreadPoolExecutor-1_0 streamlink.stream.dash audio/mp4 segment 9: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:33.001522Z)
2023-10-04 10:17:33,001.001 debug Thread-DASHStreamWriter streamlink.stream.dash audio/mp4 segment 8: completed
2023-10-04 10:17:33,009.009 debug ThreadPoolExecutor-0_0 streamlink.stream.dash video/mp4 segment 5: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:33.009335Z)
2023-10-04 10:17:33,011.011 debug Thread-DASHStreamWriter streamlink.stream.dash video/mp4 segment 4: completed
2023-10-04 10:17:33,023.023 debug ThreadPoolExecutor-1_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185958-audio.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 102846
2023-10-04 10:17:33,033.033 debug ThreadPoolExecutor-1_0 streamlink.stream.dash audio/mp4 segment 10: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:33.033628Z)
2023-10-04 10:17:33,035.035 debug ThreadPoolExecutor-0_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185954-video.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 1868840
2023-10-04 10:17:33,036.036 debug Thread-DASHStreamWriter streamlink.stream.dash audio/mp4 segment 9: completed
2023-10-04 10:17:33,061.061 debug ThreadPoolExecutor-1_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185959-audio.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 101360
2023-10-04 10:17:33,102.102 debug ThreadPoolExecutor-1_0 streamlink.stream.dash audio/mp4 segment 11: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:33.102506Z)
2023-10-04 10:17:33,102.102 debug Thread-DASHStreamWriter streamlink.stream.dash audio/mp4 segment 10: completed
2023-10-04 10:17:33,129.129 debug ThreadPoolExecutor-1_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185960-audio.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 100342
2023-10-04 10:17:33,171.171 debug ThreadPoolExecutor-1_0 streamlink.stream.dash audio/mp4 segment 12: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:33.171730Z)
2023-10-04 10:17:33,173.173 debug Thread-DASHStreamWriter streamlink.stream.dash audio/mp4 segment 11: completed
2023-10-04 10:17:33,197.197 debug ThreadPoolExecutor-1_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185961-audio.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 98520
2023-10-04 10:17:33,226.226 debug ThreadPoolExecutor-1_0 streamlink.stream.dash audio/mp4 segment 13: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:33.226648Z)
2023-10-04 10:17:33,227.227 debug Thread-DASHStreamWriter streamlink.stream.dash audio/mp4 segment 12: completed
2023-10-04 10:17:33,236.236 debug ThreadPoolExecutor-0_0 streamlink.stream.dash video/mp4 segment 6: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:33.236336Z)
2023-10-04 10:17:33,243.243 debug Thread-DASHStreamWriter streamlink.stream.dash video/mp4 segment 5: completed
2023-10-04 10:17:33,262.262 debug ThreadPoolExecutor-0_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185955-video.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 1805453
2023-10-04 10:17:33,271.271 debug ThreadPoolExecutor-1_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185962-audio.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 105987
2023-10-04 10:17:33,322.322 debug ThreadPoolExecutor-1_0 streamlink.stream.dash audio/mp4 segment 14: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:33.322592Z)
2023-10-04 10:17:33,324.324 debug Thread-DASHStreamWriter streamlink.stream.dash audio/mp4 segment 13: completed
2023-10-04 10:17:33,377.377 debug ThreadPoolExecutor-1_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185963-audio.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 104956
2023-10-04 10:17:33,430.430 debug ThreadPoolExecutor-1_0 streamlink.stream.dash audio/mp4 segment 15: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:33.430328Z)
2023-10-04 10:17:33,430.430 debug Thread-DASHStreamWriter streamlink.stream.dash audio/mp4 segment 14: completed
2023-10-04 10:17:33,442.442 debug ThreadPoolExecutor-0_0 streamlink.stream.dash video/mp4 segment 7: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:33.442022Z)
2023-10-04 10:17:33,445.445 debug Thread-DASHStreamWriter streamlink.stream.dash video/mp4 segment 6: completed
2023-10-04 10:17:33,458.458 debug ThreadPoolExecutor-1_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185964-audio.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 99766
2023-10-04 10:17:33,463.463 debug ThreadPoolExecutor-0_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185956-video.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 1776850
2023-10-04 10:17:33,509.509 debug ThreadPoolExecutor-1_0 streamlink.stream.dash audio/mp4 segment 16: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:33.509435Z)
2023-10-04 10:17:33,511.511 debug Thread-DASHStreamWriter streamlink.stream.dash audio/mp4 segment 15: completed
2023-10-04 10:17:33,633.633 debug ThreadPoolExecutor-1_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185965-audio.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 100069
2023-10-04 10:17:33,639.639 debug ThreadPoolExecutor-0_0 streamlink.stream.dash video/mp4 segment 8: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:33.639391Z)
2023-10-04 10:17:33,643.643 debug Thread-DASHStreamWriter streamlink.stream.dash video/mp4 segment 7: completed
2023-10-04 10:17:33,661.661 debug Thread-DASHStreamWriter streamlink.stream.dash audio/mp4 segment 16: completed
2023-10-04 10:17:33,664.664 debug ThreadPoolExecutor-0_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185957-video.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 1811807
2023-10-04 10:17:33,826.826 debug ThreadPoolExecutor-0_0 streamlink.stream.dash video/mp4 segment 9: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:33.826676Z)
2023-10-04 10:17:33,828.828 debug Thread-DASHStreamWriter streamlink.stream.dash video/mp4 segment 8: completed
2023-10-04 10:17:33,850.850 debug ThreadPoolExecutor-0_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185958-video.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 1451015
2023-10-04 10:17:33,994.994 debug ThreadPoolExecutor-0_0 streamlink.stream.dash video/mp4 segment 10: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:33.994051Z)
2023-10-04 10:17:33,995.995 debug Thread-DASHStreamWriter streamlink.stream.dash video/mp4 segment 9: completed
2023-10-04 10:17:34,019.019 debug ThreadPoolExecutor-0_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185959-video.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 1708892
2023-10-04 10:17:34,192.192 debug ThreadPoolExecutor-0_0 streamlink.stream.dash video/mp4 segment 11: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:34.192532Z)
2023-10-04 10:17:34,194.194 debug Thread-DASHStreamWriter streamlink.stream.dash video/mp4 segment 10: completed
2023-10-04 10:17:34,215.215 debug ThreadPoolExecutor-0_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185960-video.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 2003115
2023-10-04 10:17:34,396.396 debug ThreadPoolExecutor-0_0 streamlink.stream.dash video/mp4 segment 12: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:34.396210Z)
2023-10-04 10:17:34,399.399 debug Thread-DASHStreamWriter streamlink.stream.dash video/mp4 segment 11: completed
2023-10-04 10:17:34,421.421 debug ThreadPoolExecutor-0_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185961-video.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 1879514
2023-10-04 10:17:34,598.598 debug ThreadPoolExecutor-0_0 streamlink.stream.dash video/mp4 segment 13: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:34.598858Z)
2023-10-04 10:17:34,600.600 debug Thread-DASHStreamWriter streamlink.stream.dash video/mp4 segment 12: completed
2023-10-04 10:17:34,636.636 debug ThreadPoolExecutor-0_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185962-video.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 1966124
2023-10-04 10:17:34,813.813 debug ThreadPoolExecutor-0_0 streamlink.stream.dash video/mp4 segment 14: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:34.813721Z)
2023-10-04 10:17:34,815.815 debug Thread-DASHStreamWriter streamlink.stream.dash video/mp4 segment 13: completed
2023-10-04 10:17:34,915.915 debug ThreadPoolExecutor-0_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185963-video.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 1732372
2023-10-04 10:17:35,183.183 debug ThreadPoolExecutor-0_0 streamlink.stream.dash video/mp4 segment 15: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:35.183834Z)
2023-10-04 10:17:35,185.185 debug Thread-DASHStreamWriter streamlink.stream.dash video/mp4 segment 14: completed
2023-10-04 10:17:35,332.332 debug ThreadPoolExecutor-0_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185964-video.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 1932602
2023-10-04 10:17:35,582.582 debug ThreadPoolExecutor-0_0 streamlink.stream.dash video/mp4 segment 16: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:35.582728Z)
2023-10-04 10:17:35,587.587 debug Thread-DASHStreamWriter streamlink.stream.dash video/mp4 segment 15: completed
2023-10-04 10:17:35,679.679 debug ThreadPoolExecutor-0_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185965-video.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 1711226
2023-10-04 10:17:35,898.898 debug Thread-DASHStreamWriter streamlink.stream.dash video/mp4 segment 16: completed
2023-10-04 10:17:37,032.032 debug Thread-DASHStreamWorker streamlink.stream.dash Reloading manifest ('0', None, 'video-5')
2023-10-04 10:17:37,033.033 debug ThreadPoolExecutor-0_0 streamlink.stream.dash video/mp4 segment initialization: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:37.033701Z)
2023-10-04 10:17:37,051.051 debug Thread-DASHStreamWorker streamlink.stream.dash Reloading manifest ('0', None, 'audio-5')
2023-10-04 10:17:37,054.054 debug ThreadPoolExecutor-1_0 streamlink.stream.dash audio/mp4 segment initialization: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:37.054544Z)
2023-10-04 10:17:37,056.056 debug ThreadPoolExecutor-0_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/init-video.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 1280
2023-10-04 10:17:37,057.057 debug ThreadPoolExecutor-0_0 streamlink.stream.dash video/mp4 segment 1: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:37.057090Z)
2023-10-04 10:17:37,057.057 debug Thread-DASHStreamWriter streamlink.stream.dash video/mp4 segment initialization: completed
2023-10-04 10:17:37,076.076 debug ThreadPoolExecutor-1_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/init-audio.mp4?hdntl=exp=1696436252~acl=/*~hmac=742d6a4186669e0f5e94a3dfdb4b34ca9f7e64a0b058a651da3b51b138c7b5a2 HTTP/1.1" 200 1208
2023-10-04 10:17:37,076.076 debug ThreadPoolExecutor-1_0 streamlink.stream.dash audio/mp4 segment 1: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:37.076652Z)
2023-10-04 10:17:37,077.077 debug Thread-DASHStreamWriter streamlink.stream.dash audio/mp4 segment initialization: completed
2023-10-04 10:17:37,079.079 debug ThreadPoolExecutor-0_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185950-video.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 1745420
2023-10-04 10:17:37,100.100 debug ThreadPoolExecutor-1_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185950-audio.mp4?hdntl=exp=1696436252~acl=/*~hmac=742d6a4186669e0f5e94a3dfdb4b34ca9f7e64a0b058a651da3b51b138c7b5a2 HTTP/1.1" 200 103963
2023-10-04 10:17:37,141.141 debug ThreadPoolExecutor-1_0 streamlink.stream.dash audio/mp4 segment 2: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:37.141307Z)
2023-10-04 10:17:37,141.141 debug Thread-DASHStreamWriter streamlink.stream.dash audio/mp4 segment 1: completed
2023-10-04 10:17:37,211.211 debug ThreadPoolExecutor-1_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185951-audio.mp4?hdntl=exp=1696436252~acl=/*~hmac=742d6a4186669e0f5e94a3dfdb4b34ca9f7e64a0b058a651da3b51b138c7b5a2 HTTP/1.1" 200 100474
2023-10-04 10:17:37,212.212 debug ThreadPoolExecutor-1_0 streamlink.stream.dash audio/mp4 segment 3: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:37.212637Z)
2023-10-04 10:17:37,213.213 debug Thread-DASHStreamWriter streamlink.stream.dash audio/mp4 segment 2: completed
2023-10-04 10:17:37,227.227 debug Thread-DASHStreamWorker urllib3.connectionpool https://dcs-live-uw1.mp.lura.live:443 "GET /server/play/OV3pDTvQYW5cMWeXW/manifest.mpd?anvsid=m177610521-na549ea847f984f7defa73e3830473903 HTTP/1.1" 200 None
2023-10-04 10:17:37,247.247 debug Thread-DASHStreamWorker charset_normalizer Encoding detection: utf_8 is most likely the one.
2023-10-04 10:17:37,257.257 debug ThreadPoolExecutor-1_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185952-audio.mp4?hdntl=exp=1696436252~acl=/*~hmac=742d6a4186669e0f5e94a3dfdb4b34ca9f7e64a0b058a651da3b51b138c7b5a2 HTTP/1.1" 200 102117
2023-10-04 10:17:37,272.272 debug ThreadPoolExecutor-0_0 streamlink.stream.dash video/mp4 segment 2: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:37.272361Z)
2023-10-04 10:17:37,274.274 debug Thread-DASHStreamWriter streamlink.stream.dash video/mp4 segment 1: completed
2023-10-04 10:17:37,275.275 debug ThreadPoolExecutor-1_0 streamlink.stream.dash audio/mp4 segment 4: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:37.275781Z)
2023-10-04 10:17:37,276.276 debug Thread-DASHStreamWriter streamlink.stream.dash audio/mp4 segment 3: completed
2023-10-04 10:17:37,280.280 debug Thread-DASHStreamWorker urllib3.connectionpool https://dcs-live-uw1.mp.lura.live:443 "GET /server/play/OV3pDTvQYW5cMWeXW/manifest.mpd?anvsid=m177610521-na549ea847f984f7defa73e3830473903 HTTP/1.1" 200 None
2023-10-04 10:17:37,297.297 debug Thread-DASHStreamWorker charset_normalizer Encoding detection: utf_8 is most likely the one.
2023-10-04 10:17:37,303.303 debug ThreadPoolExecutor-1_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185953-audio.mp4?hdntl=exp=1696436252~acl=/*~hmac=742d6a4186669e0f5e94a3dfdb4b34ca9f7e64a0b058a651da3b51b138c7b5a2 HTTP/1.1" 200 99864
2023-10-04 10:17:37,305.305 debug ThreadPoolExecutor-0_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185951-video.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 1880960
2023-10-04 10:17:37,342.342 debug ThreadPoolExecutor-1_0 streamlink.stream.dash audio/mp4 segment 5: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:37.342266Z)
2023-10-04 10:17:37,342.342 debug Thread-DASHStreamWriter streamlink.stream.dash audio/mp4 segment 4: completed
2023-10-04 10:17:37,391.391 debug ThreadPoolExecutor-1_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185954-audio.mp4?hdntl=exp=1696436252~acl=/*~hmac=742d6a4186669e0f5e94a3dfdb4b34ca9f7e64a0b058a651da3b51b138c7b5a2 HTTP/1.1" 200 104877
2023-10-04 10:17:37,496.496 debug ThreadPoolExecutor-0_0 streamlink.stream.dash video/mp4 segment 3: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:37.496420Z)
2023-10-04 10:17:37,500.500 debug Thread-DASHStreamWriter streamlink.stream.dash video/mp4 segment 2: completed
2023-10-04 10:17:37,517.517 debug ThreadPoolExecutor-0_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185952-video.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 1749488
2023-10-04 10:17:37,694.694 debug ThreadPoolExecutor-1_0 streamlink.stream.dash audio/mp4 segment 6: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:37.693999Z)
2023-10-04 10:17:37,694.694 debug Thread-DASHStreamWriter streamlink.stream.dash audio/mp4 segment 5: completed
2023-10-04 10:17:37,699.699 debug ThreadPoolExecutor-0_0 streamlink.stream.dash video/mp4 segment 4: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:37.699439Z)
2023-10-04 10:17:37,702.702 debug Thread-DASHStreamWriter streamlink.stream.dash video/mp4 segment 3: completed
2023-10-04 10:17:37,716.716 debug ThreadPoolExecutor-1_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185955-audio.mp4?hdntl=exp=1696436252~acl=/*~hmac=742d6a4186669e0f5e94a3dfdb4b34ca9f7e64a0b058a651da3b51b138c7b5a2 HTTP/1.1" 200 100514
2023-10-04 10:17:37,722.722 debug ThreadPoolExecutor-0_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185953-video.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 1762684
2023-10-04 10:17:37,749.749 debug ThreadPoolExecutor-1_0 streamlink.stream.dash audio/mp4 segment 7: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:37.749239Z)
2023-10-04 10:17:37,750.750 debug Thread-DASHStreamWriter streamlink.stream.dash audio/mp4 segment 6: completed
2023-10-04 10:17:37,799.799 debug ThreadPoolExecutor-1_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185956-audio.mp4?hdntl=exp=1696436252~acl=/*~hmac=742d6a4186669e0f5e94a3dfdb4b34ca9f7e64a0b058a651da3b51b138c7b5a2 HTTP/1.1" 200 102101
2023-10-04 10:17:37,837.837 debug ThreadPoolExecutor-1_0 streamlink.stream.dash audio/mp4 segment 8: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:37.837639Z)
2023-10-04 10:17:37,837.837 debug Thread-DASHStreamWriter streamlink.stream.dash audio/mp4 segment 7: completed
2023-10-04 10:17:37,886.886 debug ThreadPoolExecutor-1_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185957-audio.mp4?hdntl=exp=1696436252~acl=/*~hmac=742d6a4186669e0f5e94a3dfdb4b34ca9f7e64a0b058a651da3b51b138c7b5a2 HTTP/1.1" 200 103303
2023-10-04 10:17:37,916.916 debug ThreadPoolExecutor-0_0 streamlink.stream.dash video/mp4 segment 5: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:37.916398Z)
2023-10-04 10:17:37,919.919 debug Thread-DASHStreamWriter streamlink.stream.dash video/mp4 segment 4: completed
2023-10-04 10:17:37,926.926 debug ThreadPoolExecutor-1_0 streamlink.stream.dash audio/mp4 segment 9: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:37.926446Z)
2023-10-04 10:17:37,926.926 debug Thread-DASHStreamWriter streamlink.stream.dash audio/mp4 segment 8: completed
2023-10-04 10:17:37,937.937 debug ThreadPoolExecutor-0_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185954-video.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 1868840
2023-10-04 10:17:37,950.950 debug ThreadPoolExecutor-1_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185958-audio.mp4?hdntl=exp=1696436252~acl=/*~hmac=742d6a4186669e0f5e94a3dfdb4b34ca9f7e64a0b058a651da3b51b138c7b5a2 HTTP/1.1" 200 102846
2023-10-04 10:17:37,994.994 debug ThreadPoolExecutor-1_0 streamlink.stream.dash audio/mp4 segment 10: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:37.994961Z)
2023-10-04 10:17:37,995.995 debug Thread-DASHStreamWriter streamlink.stream.dash audio/mp4 segment 9: completed
2023-10-04 10:17:38,042.042 debug ThreadPoolExecutor-1_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185959-audio.mp4?hdntl=exp=1696436252~acl=/*~hmac=742d6a4186669e0f5e94a3dfdb4b34ca9f7e64a0b058a651da3b51b138c7b5a2 HTTP/1.1" 200 101360
2023-10-04 10:17:38,130.130 debug ThreadPoolExecutor-0_0 streamlink.stream.dash video/mp4 segment 6: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:38.130829Z)
2023-10-04 10:17:38,132.132 debug Thread-DASHStreamWriter streamlink.stream.dash video/mp4 segment 5: completed
2023-10-04 10:17:38,154.154 debug ThreadPoolExecutor-0_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185955-video.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 1805453
2023-10-04 10:17:38,316.316 debug ThreadPoolExecutor-0_0 streamlink.stream.dash video/mp4 segment 7: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:38.316477Z)
2023-10-04 10:17:38,320.320 debug Thread-DASHStreamWriter streamlink.stream.dash video/mp4 segment 6: completed
2023-10-04 10:17:38,320.320 debug ThreadPoolExecutor-1_0 streamlink.stream.dash audio/mp4 segment 11: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:38.320806Z)
2023-10-04 10:17:38,322.322 debug Thread-DASHStreamWriter streamlink.stream.dash audio/mp4 segment 10: completed
2023-10-04 10:17:38,338.338 debug ThreadPoolExecutor-0_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185956-video.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 1776850
2023-10-04 10:17:38,345.345 debug ThreadPoolExecutor-1_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185960-audio.mp4?hdntl=exp=1696436252~acl=/*~hmac=742d6a4186669e0f5e94a3dfdb4b34ca9f7e64a0b058a651da3b51b138c7b5a2 HTTP/1.1" 200 100342
2023-10-04 10:17:38,390.390 debug ThreadPoolExecutor-1_0 streamlink.stream.dash audio/mp4 segment 12: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:38.390485Z)
2023-10-04 10:17:38,390.390 debug Thread-DASHStreamWriter streamlink.stream.dash audio/mp4 segment 11: completed
2023-10-04 10:17:38,437.437 debug ThreadPoolExecutor-1_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185961-audio.mp4?hdntl=exp=1696436252~acl=/*~hmac=742d6a4186669e0f5e94a3dfdb4b34ca9f7e64a0b058a651da3b51b138c7b5a2 HTTP/1.1" 200 98520
2023-10-04 10:17:38,459.459 debug ThreadPoolExecutor-1_0 streamlink.stream.dash audio/mp4 segment 13: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:38.459783Z)
2023-10-04 10:17:38,460.460 debug Thread-DASHStreamWriter streamlink.stream.dash audio/mp4 segment 12: completed
2023-10-04 10:17:38,508.508 debug ThreadPoolExecutor-1_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185962-audio.mp4?hdntl=exp=1696436252~acl=/*~hmac=742d6a4186669e0f5e94a3dfdb4b34ca9f7e64a0b058a651da3b51b138c7b5a2 HTTP/1.1" 200 105987
2023-10-04 10:17:38,517.517 debug ThreadPoolExecutor-1_0 streamlink.stream.dash audio/mp4 segment 14: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:38.517094Z)
2023-10-04 10:17:38,517.517 debug Thread-DASHStreamWriter streamlink.stream.dash audio/mp4 segment 13: completed
2023-10-04 10:17:38,529.529 debug ThreadPoolExecutor-0_0 streamlink.stream.dash video/mp4 segment 8: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:38.529086Z)
2023-10-04 10:17:38,530.530 debug Thread-DASHStreamWriter streamlink.stream.dash video/mp4 segment 7: completed
2023-10-04 10:17:38,538.538 debug ThreadPoolExecutor-1_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185963-audio.mp4?hdntl=exp=1696436252~acl=/*~hmac=742d6a4186669e0f5e94a3dfdb4b34ca9f7e64a0b058a651da3b51b138c7b5a2 HTTP/1.1" 200 104956
2023-10-04 10:17:38,546.546 debug ThreadPoolExecutor-1_0 streamlink.stream.dash audio/mp4 segment 15: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:38.546356Z)
2023-10-04 10:17:38,546.546 debug Thread-DASHStreamWriter streamlink.stream.dash audio/mp4 segment 14: completed
2023-10-04 10:17:38,551.551 debug ThreadPoolExecutor-0_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185957-video.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 1811807
2023-10-04 10:17:38,571.571 debug ThreadPoolExecutor-1_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185964-audio.mp4?hdntl=exp=1696436252~acl=/*~hmac=742d6a4186669e0f5e94a3dfdb4b34ca9f7e64a0b058a651da3b51b138c7b5a2 HTTP/1.1" 200 99766
2023-10-04 10:17:38,614.614 debug ThreadPoolExecutor-1_0 streamlink.stream.dash audio/mp4 segment 16: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:38.614774Z)
2023-10-04 10:17:38,616.616 debug Thread-DASHStreamWriter streamlink.stream.dash audio/mp4 segment 15: completed
2023-10-04 10:17:38,637.637 debug ThreadPoolExecutor-1_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185965-audio.mp4?hdntl=exp=1696436252~acl=/*~hmac=742d6a4186669e0f5e94a3dfdb4b34ca9f7e64a0b058a651da3b51b138c7b5a2 HTTP/1.1" 200 100069
2023-10-04 10:17:38,682.682 debug Thread-DASHStreamWriter streamlink.stream.dash audio/mp4 segment 16: completed
2023-10-04 10:17:38,747.747 debug ThreadPoolExecutor-0_0 streamlink.stream.dash video/mp4 segment 9: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:38.747640Z)
2023-10-04 10:17:38,749.749 debug Thread-DASHStreamWriter streamlink.stream.dash video/mp4 segment 8: completed
2023-10-04 10:17:38,772.772 debug ThreadPoolExecutor-0_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185958-video.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 1451015
2023-10-04 10:17:38,901.901 debug ThreadPoolExecutor-0_0 streamlink.stream.dash video/mp4 segment 10: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:38.901147Z)
2023-10-04 10:17:38,904.904 debug Thread-DASHStreamWriter streamlink.stream.dash video/mp4 segment 9: completed
2023-10-04 10:17:38,922.922 debug ThreadPoolExecutor-0_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185959-video.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 1708892
2023-10-04 10:17:39,094.094 debug ThreadPoolExecutor-0_0 streamlink.stream.dash video/mp4 segment 11: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:39.093988Z)
2023-10-04 10:17:39,095.095 debug Thread-DASHStreamWriter streamlink.stream.dash video/mp4 segment 10: completed
2023-10-04 10:17:39,122.122 debug ThreadPoolExecutor-0_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185960-video.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 2003115
2023-10-04 10:17:39,304.304 debug ThreadPoolExecutor-0_0 streamlink.stream.dash video/mp4 segment 12: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:39.304407Z)
2023-10-04 10:17:39,309.309 debug Thread-DASHStreamWriter streamlink.stream.dash video/mp4 segment 11: completed
2023-10-04 10:17:39,327.327 debug ThreadPoolExecutor-0_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185961-video.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 1879514
2023-10-04 10:17:39,495.495 debug ThreadPoolExecutor-0_0 streamlink.stream.dash video/mp4 segment 13: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:39.495373Z)
2023-10-04 10:17:39,497.497 debug Thread-DASHStreamWriter streamlink.stream.dash video/mp4 segment 12: completed
2023-10-04 10:17:39,518.518 debug ThreadPoolExecutor-0_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185962-video.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 1966124
2023-10-04 10:17:39,696.696 debug ThreadPoolExecutor-0_0 streamlink.stream.dash video/mp4 segment 14: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:39.696014Z)
2023-10-04 10:17:39,700.700 debug Thread-DASHStreamWriter streamlink.stream.dash video/mp4 segment 13: completed
2023-10-04 10:17:39,722.722 debug ThreadPoolExecutor-0_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185963-video.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 1732372
2023-10-04 10:17:39,876.876 debug ThreadPoolExecutor-0_0 streamlink.stream.dash video/mp4 segment 15: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:39.876885Z)
2023-10-04 10:17:39,879.879 debug Thread-DASHStreamWriter streamlink.stream.dash video/mp4 segment 14: completed
2023-10-04 10:17:39,901.901 debug ThreadPoolExecutor-0_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185964-video.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 1932602
2023-10-04 10:17:40,090.090 debug ThreadPoolExecutor-0_0 streamlink.stream.dash video/mp4 segment 16: downloading (2023-10-04T10:16:26.000000Z / 2023-10-04T10:17:40.090599Z)
2023-10-04 10:17:40,093.093 debug Thread-DASHStreamWriter streamlink.stream.dash video/mp4 segment 15: completed
2023-10-04 10:17:40,118.118 debug ThreadPoolExecutor-0_0 urllib3.connectionpool https://video-live.dpgmedia.net:443 "GET /live/ephemeral/Py1vlQKiKkE3miEiwpD10CMNeZELWKVq/vtm4-x-dist/4792k/ugSxF45MOHE/178185/segment_178185965-video.mp4?hdntl=exp=1696436251~acl=/*~hmac=2d5245e3f99f770856f92b33d2c6c8359bfc814f51848980a7d9bf313a5b9e68 HTTP/1.1" 200 1711226
2023-10-04 10:17:40,271.271 debug Thread-DASHStreamWriter streamlink.stream.dash video/mp4 segment 16: completed
```
| The full manifest can be found here: https://pastebin.com/mCHDZNUB
Streamlink currently doesn't support dynamic DASH manifests with `SegmentList`s.
The yielded segment list doesn't keep track of the last segment position, so after a manifest reload, it starts from anew.
This is also the reason why it starts from the beginning, rather than from the live position with an offset.
> I've looked at the code, and it seems the whole playlist is reloaded in `stream.dash.reload()` and there is no code to filter out already downloaded segments.
It's only implemented for `SegmentTemplate`s with timelines:
https://github.com/streamlink/streamlink/blame/6.2.1/src/streamlink/stream/dash_manifest.py#L887-L919
| 2023-11-07T16:38:57 |
streamlink/streamlink | 5,673 | streamlink__streamlink-5673 | [
"5672"
] | d8e677bc1a6408cace4f6b584091e41f778b94c5 | diff --git a/src/streamlink/plugins/youtube.py b/src/streamlink/plugins/youtube.py
--- a/src/streamlink/plugins/youtube.py
+++ b/src/streamlink/plugins/youtube.py
@@ -335,8 +335,10 @@ def _get_data_from_api(self, res):
@staticmethod
def _data_video_id(data):
- if data:
- for videoRenderer in search_dict(data, "videoRenderer"):
+ if not data:
+ return None
+ for key in ("videoRenderer", "gridVideoRenderer"):
+ for videoRenderer in search_dict(data, key):
videoId = videoRenderer.get("videoId")
if videoId is not None:
return videoId
| plugins.youtube: Could not find videoId on channel page but there's a live-stream available
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
streamlink 6.3.1
### Description
I've been getting the following error since yesterday on multiple youtube channels that actually have at least one live-stream available but the plugin seems to be unable to find it:
```
[plugins.youtube][error] Could not find videoId on channel page
```
Here's a non-exhaustive list of channels that I've been able to reproduce this error:
- https://www.youtube.com/@ABCNews
- https://www.youtube.com/user/ABCNews
- https://www.youtube.com/@lanacion (added to the debug log)
- https://www.youtube.com/user/LaNacionTV
- https://www.youtube.com/@LofiGirl (added to the debug log)
- https://www.youtube.com/@France24_en
Please check the debug log for details.
Of note, there are a few channels that continue to work just fine, namely:
- https://www.youtube.com/@jazzbossacollection7548
- https://www.youtube.com/@recordnews (added to the debug log)
### Debug log
```text
streamlink --loglevel debug https://www.youtube.com/@LofiGirl
[cli][debug] OS: Linux-6.1.0-13-amd64-x86_64-with-glibc2.36
[cli][debug] Python: 3.11.2
[cli][debug] OpenSSL: OpenSSL 3.0.11 19 Sep 2023
[cli][debug] Streamlink: 6.3.1
[cli][debug] Dependencies:
[cli][debug] certifi: 2022.9.24
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.2
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.18.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.28.1
[cli][debug] trio: 0.22.2
[cli][debug] trio-websocket: 0.10.3
[cli][debug] typing-extensions: 4.7.1
[cli][debug] urllib3: 1.26.12
[cli][debug] websocket-client: 1.6.4
[cli][debug] Arguments:
[cli][debug] url=https://www.youtube.com/@LofiGirl
[cli][debug] --loglevel=debug
[cli][info] Found matching plugin youtube for URL https://www.youtube.com/@LofiGirl
[plugins.youtube][error] Could not find videoId on channel page
error: No playable streams found on this URL: https://www.youtube.com/@LofiGirl
---
streamlink --loglevel debug https://www.youtube.com/@lanacion
[cli][debug] OS: Linux-6.1.0-13-amd64-x86_64-with-glibc2.36
[cli][debug] Python: 3.11.2
[cli][debug] OpenSSL: OpenSSL 3.0.11 19 Sep 2023
[cli][debug] Streamlink: 6.3.1
[cli][debug] Dependencies:
[cli][debug] certifi: 2022.9.24
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.2
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.18.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.28.1
[cli][debug] trio: 0.22.2
[cli][debug] trio-websocket: 0.10.3
[cli][debug] typing-extensions: 4.7.1
[cli][debug] urllib3: 1.26.12
[cli][debug] websocket-client: 1.6.4
[cli][debug] Arguments:
[cli][debug] url=https://www.youtube.com/@lanacion
[cli][debug] --loglevel=debug
[cli][info] Found matching plugin youtube for URL https://www.youtube.com/@lanacion
[plugins.youtube][error] Could not find videoId on channel page
error: No playable streams found on this URL: https://www.youtube.com/@lanacion
---
streamlink --loglevel debug https://www.youtube.com/@recordnews
[cli][debug] OS: Linux-6.1.0-13-amd64-x86_64-with-glibc2.36
[cli][debug] Python: 3.11.2
[cli][debug] OpenSSL: OpenSSL 3.0.11 19 Sep 2023
[cli][debug] Streamlink: 6.3.1
[cli][debug] Dependencies:
[cli][debug] certifi: 2022.9.24
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.2
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.18.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.28.1
[cli][debug] trio: 0.22.2
[cli][debug] trio-websocket: 0.10.3
[cli][debug] typing-extensions: 4.7.1
[cli][debug] urllib3: 1.26.12
[cli][debug] websocket-client: 1.6.4
[cli][debug] Arguments:
[cli][debug] url=https://www.youtube.com/@recordnews
[cli][debug] --loglevel=debug
[cli][info] Found matching plugin youtube for URL https://www.youtube.com/@recordnews
[plugins.youtube][debug] Using video ID: QSGgKI9N9Mk
[plugins.youtube][debug] This video is live.
[utils.l10n][debug] Language code: en_US
Available streams: 144p (worst), 240p, 360p, 480p, 720p, 1080p (best)
```
| #5434
The `ytInitialData` JSON format has once again changed...
This should fix it, but I will have to make further tests:
```diff
diff --git a/src/streamlink/plugins/youtube.py b/src/streamlink/plugins/youtube.py
index a85290c5..9824f16b 100644
--- a/src/streamlink/plugins/youtube.py
+++ b/src/streamlink/plugins/youtube.py
@@ -335,8 +335,10 @@ class YouTube(Plugin):
@staticmethod
def _data_video_id(data):
- if data:
- for videoRenderer in search_dict(data, "videoRenderer"):
+ if not data:
+ return None
+ for key in ("videoRenderer", "gridVideoRenderer"):
+ for videoRenderer in search_dict(data, key):
videoId = videoRenderer.get("videoId")
if videoId is not None:
return videoId
```
```
$ streamlink -l debug https://www.youtube.com/@LofiGirl
[cli][debug] OS: Linux-6.6.1-1-git-x86_64-with-glibc2.38
[cli][debug] Python: 3.11.5
[cli][debug] OpenSSL: OpenSSL 3.1.4 24 Oct 2023
[cli][debug] Streamlink: 6.3.1+25.gd8e677bc.dirty
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.7.22
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.3
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.19.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.22.2
[cli][debug] trio-websocket: 0.11.1
[cli][debug] typing-extensions: 4.8.0
[cli][debug] urllib3: 2.0.6
[cli][debug] websocket-client: 1.6.4
[cli][debug] Arguments:
[cli][debug] url=https://www.youtube.com/@LofiGirl
[cli][debug] --loglevel=debug
[cli][debug] --player=mpv
[cli][info] Found matching plugin youtube for URL https://www.youtube.com/@LofiGirl
[plugins.youtube][debug] consent target: https://consent.youtube.com/save
[plugins.youtube][debug] consent data: gl, m, app, pc, continue, x, bl, hl, src, cm, set_ytc, set_apyt, set_eom
[plugins.youtube][debug] Using video ID: jfKfPfyJRdk
[plugins.youtube][debug] This video is live.
[utils.l10n][debug] Language code: en_US
Available streams: 144p (worst), 240p, 360p, 480p, 720p, 1080p (best)
``` | 2023-11-18T16:40:39 |
|
streamlink/streamlink | 5,682 | streamlink__streamlink-5682 | [
"5681"
] | 0048c67772a8030a953e784ddc91ccf360ef42eb | diff --git a/src/streamlink/compat.py b/src/streamlink/compat.py
--- a/src/streamlink/compat.py
+++ b/src/streamlink/compat.py
@@ -2,11 +2,22 @@
import sys
+# compatibility import of charset_normalizer/chardet via requests<3.0
+try:
+ from requests.compat import chardet as charset_normalizer # type: ignore
+except ImportError: # pragma: no cover
+ import charset_normalizer
+
+
is_darwin = sys.platform == "darwin"
is_win32 = os.name == "nt"
+detect_encoding = charset_normalizer.detect
+
+
__all__ = [
"is_darwin",
"is_win32",
+ "detect_encoding",
]
diff --git a/src/streamlink/utils/parse.py b/src/streamlink/utils/parse.py
--- a/src/streamlink/utils/parse.py
+++ b/src/streamlink/utils/parse.py
@@ -4,6 +4,7 @@
from lxml.etree import HTML, XML
+from streamlink.compat import detect_encoding
from streamlink.plugin import PluginError
@@ -51,7 +52,21 @@ def parse_html(
- Removes XML declarations of invalid XHTML5 documents
- Wraps errors in custom exception with a snippet of the data in the message
"""
- if isinstance(data, str) and data.lstrip().startswith("<?xml"):
+ # strip XML text declarations from XHTML5 documents which were incorrectly defined as HTML5
+ is_bytes = isinstance(data, bytes)
+ if data and data.lstrip()[:5].lower() == (b"<?xml" if is_bytes else "<?xml"):
+ if is_bytes:
+ # get the document's encoding using the "encoding" attribute value of the XML text declaration
+ match = re.match(rb"^\s*<\?xml\s.*?encoding=(?P<q>[\'\"])(?P<encoding>.+?)(?P=q).*?\?>", data, re.IGNORECASE)
+ if match:
+ encoding_value = detect_encoding(match["encoding"])["encoding"]
+ encoding = match["encoding"].decode(encoding_value)
+ else:
+ # no "encoding" attribute: try to figure out encoding from the document's content
+ encoding = detect_encoding(data)["encoding"]
+
+ data = data.decode(encoding)
+
data = re.sub(r"^\s*<\?xml.+?\?>", "", data)
return _parse(HTML, data, name, exception, schema, *args, **kwargs)
| diff --git a/tests/utils/test_parse.py b/tests/utils/test_parse.py
--- a/tests/utils/test_parse.py
+++ b/tests/utils/test_parse.py
@@ -74,31 +74,93 @@ def test_parse_xml_entities(self):
assert actual.tag == expected.tag
assert actual.attrib == expected.attrib
- def test_parse_xml_encoding(self):
- tree = parse_xml("""<?xml version="1.0" encoding="UTF-8"?><test>Γ€</test>""")
- assert tree.xpath(".//text()") == ["Γ€"]
- tree = parse_xml("""<test>Γ€</test>""")
- assert tree.xpath(".//text()") == ["Γ€"]
- tree = parse_xml(b"""<?xml version="1.0" encoding="UTF-8"?><test>\xC3\xA4</test>""")
- assert tree.xpath(".//text()") == ["Γ€"]
- tree = parse_xml(b"""<test>\xC3\xA4</test>""")
- assert tree.xpath(".//text()") == ["Γ€"]
-
- def test_parse_html_encoding(self):
- tree = parse_html("""<!DOCTYPE html><html><head><meta charset="utf-8"/></head><body>Γ€</body></html>""")
- assert tree.xpath(".//body/text()") == ["Γ€"]
- tree = parse_html("""<!DOCTYPE html><html><body>Γ€</body></html>""")
- assert tree.xpath(".//body/text()") == ["Γ€"]
- tree = parse_html(b"""<!DOCTYPE html><html><meta charset="utf-8"/><body>\xC3\xA4</body></html>""")
- assert tree.xpath(".//body/text()") == ["Γ€"]
- tree = parse_html(b"""<!DOCTYPE html><html><body>\xC3\xA4</body></html>""")
- assert tree.xpath(".//body/text()") == ["ΓΒ€"]
-
- def test_parse_html_xhtml5(self):
- tree = parse_html("""<?xml version="1.0" encoding="UTF-8"?><!DOCTYPE html><html><body>Γ€?></body></html>""")
- assert tree.xpath(".//body/text()") == ["Γ€?>"]
- tree = parse_html(b"""<?xml version="1.0" encoding="UTF-8"?><!DOCTYPE html><html><body>\xC3\xA4?></body></html>""")
- assert tree.xpath(".//body/text()") == ["Γ€?>"]
+ @pytest.mark.parametrize(("content", "expected"), [
+ pytest.param(
+ """<?xml version="1.0" encoding="UTF-8"?><test>Γ€</test>""",
+ "Γ€",
+ id="string-utf-8",
+ ),
+ pytest.param(
+ """<test>Γ€</test>""",
+ "Γ€",
+ id="string-unknown",
+ ),
+ pytest.param(
+ b"""<?xml version="1.0" encoding="UTF-8"?><test>\xC3\xA4</test>""",
+ "Γ€",
+ id="bytes-utf-8",
+ ),
+ pytest.param(
+ b"""<?xml version="1.0" encoding="ISO-8859-1"?><test>\xE4</test>""",
+ "Γ€",
+ id="bytes-iso-8859-1",
+ ),
+ pytest.param(
+ b"""<test>\xC3\xA4</test>""",
+ "Γ€",
+ id="bytes-unknown",
+ ),
+ ])
+ def test_parse_xml_encoding(self, content, expected):
+ tree = parse_xml(content)
+ assert tree.xpath(".//text()") == [expected]
+
+ @pytest.mark.parametrize(("content", "expected"), [
+ pytest.param(
+ """<!DOCTYPE html><html><head><meta charset="utf-8"/></head><body>Γ€</body></html>""",
+ "Γ€",
+ id="string-utf-8",
+ ),
+ pytest.param(
+ """<!DOCTYPE html><html><body>Γ€</body></html>""",
+ "Γ€",
+ id="string-unknown",
+ ),
+ pytest.param(
+ b"""<!DOCTYPE html><html><head><meta charset="utf-8"/></head><body>\xC3\xA4</body></html>""",
+ "Γ€",
+ id="bytes-utf-8",
+ ),
+ pytest.param(
+ b"""<!DOCTYPE html><html><head><meta charset="ISO-8859-1"/></head><body>\xE4</body></html>""",
+ "Γ€",
+ id="bytes-iso-8859-1",
+ ),
+ pytest.param(
+ b"""<!DOCTYPE html><html><body>\xC3\xA4</body></html>""",
+ "ΓΒ€",
+ id="bytes-unknown",
+ ),
+ ])
+ def test_parse_html_encoding(self, content, expected):
+ tree = parse_html(content)
+ assert tree.xpath(".//body/text()") == [expected]
+
+ @pytest.mark.parametrize(("content", "expected"), [
+ pytest.param(
+ """<?xml version="1.0" encoding="UTF-8"?><!DOCTYPE html><html><body>Γ€?></body></html>""",
+ "Γ€?>",
+ id="string",
+ ),
+ pytest.param(
+ b"""<?xml version="1.0" encoding="UTF-8"?><!DOCTYPE html><html><body>\xC3\xA4?></body></html>""",
+ "Γ€?>",
+ id="bytes-utf-8",
+ ),
+ pytest.param(
+ b"""<?xml version="1.0" encoding="ISO-8859-1"?><!DOCTYPE html><html><body>\xE4?></body></html>""",
+ "Γ€?>",
+ id="bytes-iso-8859-1",
+ ),
+ pytest.param(
+ b"""<?xml version="1.0"?><!DOCTYPE html><html><body>\xC3\xA4?></body></html>""",
+ "Γ€?>",
+ id="bytes-unknown",
+ ),
+ ])
+ def test_parse_html_xhtml5(self, content, expected):
+ tree = parse_html(content)
+ assert tree.xpath(".//body/text()") == [expected]
def test_parse_qsd(self):
assert parse_qsd("test=1&foo=bar", schema=validate.Schema({"test": str, "foo": "bar"})) == {"test": "1", "foo": "bar"}
| tests: `tests.utils.test_parse.TestUtilsParse.test_parse_html_xhtml5` failure with libxml2 2.12.0
### Checklist
- [X] This is a bug report and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed bug reports](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22bug%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
6.4.0
### Description
While trying to build the Arch package, I run into this test failure, which seems to be an encoding problem if I'm not mistaken.
### Debug log
```text
=================================== FAILURES ===================================
____________________ TestUtilsParse.test_parse_html_xhtml5 _____________________
self = <tests.utils.test_parse.TestUtilsParse object at 0x7fa710eb4b90>
def test_parse_html_xhtml5(self):
tree = parse_html("""<?xml version="1.0" encoding="UTF-8"?><!DOCTYPE html><html><body>Γ€?></body></html>""")
assert tree.xpath(".//body/text()") == ["Γ€?>"]
tree = parse_html(b"""<?xml version="1.0" encoding="UTF-8"?><!DOCTYPE html><html><body>\xC3\xA4?></body></html>""")
> assert tree.xpath(".//body/text()") == ["Γ€?>"]
E AssertionError: assert ['ΓΒ€?>'] == ['Γ€?>']
E At index 0 diff: 'ΓΒ€?>' != 'Γ€?>'
E Use -v to get more diff
tests/utils/test_parse.py:101: AssertionError
```
| This must've been caused by the recent `libxml2` bump, which is what drives `lxml` when parsing the XML/HTML:
https://gitlab.archlinux.org/archlinux/packaging/packages/libxml2/-/commits/main
Just skip this test for now...
This issue doesn't come up in our tests here, because the lxml wheel that's being used is statically linked and bundles an older libxml2 version.
- https://github.com/streamlink/streamlink/actions/runs/6946787020/job/18899101200#step:5:155
- https://github.com/lxml/lxml/blob/lxml-4.9.3/.github/workflows/wheels.yml#L118-L119
- https://github.com/lxml/lxml/blob/lxml-4.9.3/.github/workflows/wheels.yml#L128-L130
```
$ pacman -Q libxml2
libxml2 2.12.0-1
$ pip install -U --force-reinstall --no-binary=lxml lxml
Collecting lxml
Downloading lxml-4.9.3.tar.gz (3.6 MB)
ββββββββββββββββββββββββββββββββββββββββ 3.6/3.6 MB 16.5 MB/s eta 0:00:00
Preparing metadata (setup.py) ... done
Building wheels for collected packages: lxml
Building wheel for lxml (setup.py) ... done
Created wheel for lxml: filename=lxml-4.9.3-cp312-cp312-linux_x86_64.whl size=8312657 sha256=918c4f93f4296899f347d95ae8fbc0bd6b456d9d02fb61d40f124ebfb2983655
Stored in directory: /home/basti/.cache/pip/wheels/ff/81/cb/e7f1a809ccebd4db07bb3fc6a3039fc9b1bd44ae0409b3ae23
Successfully built lxml
Installing collected packages: lxml
Attempting uninstall: lxml
Found existing installation: lxml 4.9.3
Uninstalling lxml-4.9.3:
Successfully uninstalled lxml-4.9.3
Successfully installed lxml-4.9.3
$ pytest -q --disable-warnings tests/utils/test_parse.py::TestUtilsParse::test_parse_html_xhtml5
F [100%]
=================================================================== FAILURES ===================================================================
____________________________________________________ TestUtilsParse.test_parse_html_xhtml5 _____________________________________________________
self = <tests.utils.test_parse.TestUtilsParse object at 0x7ffa72760bf0>
def test_parse_html_xhtml5(self):
tree = parse_html("""<?xml version="1.0" encoding="UTF-8"?><!DOCTYPE html><html><body>Γ€?></body></html>""")
assert tree.xpath(".//body/text()") == ["Γ€?>"]
tree = parse_html(b"""<?xml version="1.0" encoding="UTF-8"?><!DOCTYPE html><html><body>\xC3\xA4?></body></html>""")
> assert tree.xpath(".//body/text()") == ["Γ€?>"]
E AssertionError: assert ['ΓΒ€?>'] == ['Γ€?>']
E At index 0 diff: 'ΓΒ€?>' != 'Γ€?>'
E Use -v to get more diff
tests/utils/test_parse.py:101: AssertionError
=========================================================== short test summary info ============================================================
FAILED tests/utils/test_parse.py::TestUtilsParse::test_parse_html_xhtml5 - AssertionError: assert ['ΓΒ€?>'] == ['Γ€?>']
1 failed, 1 warning in 0.08s
```
This test was added in #4210 because of invalid doctype definitions on some websites. Explanation here: https://github.com/streamlink/streamlink/issues/4209#issuecomment-980173868
The function can receive two different kinds of inputs, `str` and `bytes`.
If it's a `str` object, then the encoding is already determined, so the unnecessary XML text declaration gets removed by our `parse_html()` wrapper and `lxml` will simply parse the remaining string as HTML.
When the input is a `bytes` object however, the encoding needs to be figured out by the parser, so the `parse_html()` wrapper doesn't remove the XML text declaration. The test defines a simple XML document with UTF-8 encoding (as `bytes`), but this apparently doesn't get parsed correctly anymore in the recent `libxml2` version, so the test's `b"\xC3\xA4"` gets decoded using ISO 8859-1, which results in `ΓΒ€` instead of `Γ€`. As explained in the linked PR/issue, it's an invalid doctype, so `libxml2` now does the correct thing by ignoring the XML text declaration when parsing the rest of the document, which is supposedly HTML.
I'll have to think about how to solve this between the two libxml2 versions.
As said, please ignore the test for now when using `libxml2>=2.12.0`:
```
pytest --deselect tests/utils/test_parse.py::TestUtilsParse::test_parse_html_xhtml5
``` | 2023-11-21T19:18:19 |
streamlink/streamlink | 5,686 | streamlink__streamlink-5686 | [
"5685"
] | 0dda8ccfcbaa79035d0c60a86dac2faae5089901 | diff --git a/src/streamlink/plugins/ssh101.py b/src/streamlink/plugins/ssh101.py
--- a/src/streamlink/plugins/ssh101.py
+++ b/src/streamlink/plugins/ssh101.py
@@ -1,5 +1,5 @@
"""
-$description Global live streaming platform.
+$description Global live-streaming platform.
$url ssh101.com
$type live
"""
@@ -21,8 +21,8 @@
class SSH101(Plugin):
def _get_streams(self):
hls_url = self.session.http.get(self.url, schema=validate.Schema(
- validate.parse_html(),
- validate.xml_xpath_string(".//source[contains(@src,'.m3u8')]/@src"),
+ re.compile(r"src:\s*(?P<q>['\"])(?P<url>https://\S+\.m3u8)(?P=q)"),
+ validate.any(None, validate.get("url")),
))
if not hls_url:
return
@@ -32,7 +32,7 @@ def _get_streams(self):
log.error("This stream is currently offline")
return
- return {"live": HLSStream(self.session, hls_url)}
+ return HLSStream.parse_variant_playlist(self.session, hls_url)
__plugin__ = SSH101
| plugins.ssh101: No playable streams found on this URL
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
6.4.1
### Description
I always "No playable streams found on this URL"
### Debug log
```text
streamlink --loglevel=debug https://ssh101.com/securelive/index.php?id=conectatvmx
[cli][debug] OS: Linux-5.15.116-1-pve-x86_64-with
[cli][debug] Python: 3.11.6
[cli][debug] OpenSSL: OpenSSL 3.1.4 24 Oct 2023
[cli][debug] Streamlink: 6.4.1
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.7.22
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.3
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.19.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.21.0
[cli][debug] trio-websocket: 0.11.1
[cli][debug] typing-extensions: 4.8.0
[cli][debug] urllib3: 1.26.18
[cli][debug] websocket-client: 1.6.4
[cli][debug] Arguments:
[cli][debug] url=https://ssh101.com/securelive/index.php?id=conectatvmx
[cli][debug] --loglevel=debug
[cli][info] Found matching plugin ssh101 for URL https://ssh101.com/securelive/index.php?id=conectatvmx
error: No playable streams found on this URL: https://ssh101.com/securelive/index.php?id=conectatvmx
```
| 2023-11-24T20:57:01 |
||
streamlink/streamlink | 5,690 | streamlink__streamlink-5690 | [
"5684"
] | adbb942911ee2dd1cee9962abb2079d043aac31a | diff --git a/src/streamlink/plugins/piczel.py b/src/streamlink/plugins/piczel.py
--- a/src/streamlink/plugins/piczel.py
+++ b/src/streamlink/plugins/piczel.py
@@ -19,7 +19,7 @@
))
class Piczel(Plugin):
_URL_STREAMS = "https://piczel.tv/api/streams"
- _URL_HLS = "https://piczel.tv/hls/{id}/index.m3u8"
+ _URL_HLS = "https://playback.piczel.tv/live/{id}/llhls.m3u8?_HLS_legacy=YES"
def _get_streams(self):
channel = self.match.group("channel")
@@ -57,7 +57,7 @@ def _get_streams(self):
if not is_live:
return
- return {"live": HLSStream(self.session, self._URL_HLS.format(id=self.id))}
+ return HLSStream.parse_variant_playlist(self.session, self._URL_HLS.format(id=self.id))
__plugin__ = Piczel
| plugins.piczel: HLS URLs have changed
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
streamlink 6.4.1
### Description
Streams on Piczel have been broken since ~October 29th. Tested on the latest git master (0dda8ccf). Presumably Piczel rolled out a new HLS streaming setup that has changed the URLs. Streams result in the following error (note, the streamer in this example is marked NSFW):
```
[stream.hls][error] Unable to open URL: https://piczel.tv/hls/61316/index.m3u8 (502 Server Error: Bad Gateway for url: https://piczel.tv/hls/61316/index.m3u8)
[cli][error] Try 1/1: Could not open stream <HLSStream ['hls', 'https://piczel.tv/hls/61316/index.m3u8']> (No data returned from stream)
error: Could not open stream <HLSStream ['hls', 'https://piczel.tv/hls/61316/index.m3u8']>, tried 1 times, exiting
[cli][info] Closing currently open stream...
```
I took a quick glance at the current website version, and I see the following URLs in use now:
https://playback.piczel.tv/live/61316/llhls.m3u8?_HLS_legacy=YES
Which supplies separate audio and video streams, like so:
https://playback.piczel.tv/live/61316/chunklist_1_audio_61316_llhls.m3u8?_HLS_legacy=YES
https://playback.piczel.tv/live/61316/chunklist_0_video_61316_llhls.m3u8?_HLS_legacy=YES
I tried swapping those URLs into MuxedHLSStream, but it only played one chunk before exiting. I didn't see any other plugins using MuxedHLSStream in the current git master, so I'm not sure if I was just using it incorrectly or if there's something more complex going on.
### Debug log
```text
$ streamlink https://piczel.tv/watch/ranon best --loglevel debug ### NOTE: Streamer is marked as NSFW!
[cli][debug] OS: Linux-6.6.1-arch1-1-x86_64-with-glibc2.38
[cli][debug] Python: 3.11.6
[cli][debug] OpenSSL: OpenSSL 3.1.4 24 Oct 2023
[cli][debug] Streamlink: 6.4.1
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.11.17
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.2
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.19.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.23.1
[cli][debug] trio-websocket: 0.11.1
[cli][debug] typing-extensions: 4.8.0
[cli][debug] urllib3: 1.26.18
[cli][debug] websocket-client: 1.6.4
[cli][debug] Arguments:
[cli][debug] url=https://piczel.tv/watch/ranon
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][info] Found matching plugin piczel for URL https://piczel.tv/watch/ranon
[cli][info] Available streams: live (worst, best)
[cli][info] Opening stream: live (hls)
[cli][info] Starting player: /usr/bin/vlc
[stream.hls][debug] Reloading playlist
[cli][debug] Pre-buffering 8192 bytes
[stream.hls][error] Unable to open URL: https://piczel.tv/hls/61316/index.m3u8 (502 Server Error: Bad Gateway for url: https://piczel.tv/hls/61316/index.m3u8)
[stream.segmented][debug] Closing worker thread
[stream.segmented][debug] Closing writer thread
[cli][error] Try 1/1: Could not open stream <HLSStream ['hls', 'https://piczel.tv/hls/61316/index.m3u8']> (No data returned from stream)
error: Could not open stream <HLSStream ['hls', 'https://piczel.tv/hls/61316/index.m3u8']>, tried 1 times, exiting
[cli][info] Closing currently open stream...
```
| `/live/{id}/llhls.m3u8?_HLS_legacy=YES` is a multivariant playlist. The plugin previously only required direct media playlists, not multivariant playlists, hence the direct `HLSStream` class instantiation instead of the `HLSStream.parse_variant_playlist()` classmethod call.
`HLSStream.parse_variant_playlist()` automatically returns `MuxedHLSStream` instances if multiple media streams need to be muxed.
```diff
diff --git a/src/streamlink/plugins/piczel.py b/src/streamlink/plugins/piczel.py
index 80687e21..07df4c37 100644
--- a/src/streamlink/plugins/piczel.py
+++ b/src/streamlink/plugins/piczel.py
@@ -19,7 +19,7 @@ from streamlink.stream.hls import HLSStream
))
class Piczel(Plugin):
_URL_STREAMS = "https://piczel.tv/api/streams"
- _URL_HLS = "https://piczel.tv/hls/{id}/index.m3u8"
+ _URL_HLS = "https://playback.piczel.tv/live/{id}/llhls.m3u8?_HLS_legacy=YES"
def _get_streams(self):
channel = self.match.group("channel")
@@ -57,7 +57,7 @@ class Piczel(Plugin):
if not is_live:
return
- return {"live": HLSStream(self.session, self._URL_HLS.format(id=self.id))}
+ return HLSStream.parse_variant_playlist(self.session, self._URL_HLS.format(id=self.id))
__plugin__ = Piczel
```
```
$ streamlink -l debug 'https://piczel.tv/watch/Feretta'
[cli][debug] OS: Linux-6.6.2-1-git-x86_64-with-glibc2.38
[cli][debug] Python: 3.11.6
[cli][debug] OpenSSL: OpenSSL 3.1.4 24 Oct 2023
[cli][debug] Streamlink: 6.4.1+0.g0dda8ccf.dirty
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.11.17
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.3
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.19.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.23.1
[cli][debug] trio-websocket: 0.11.1
[cli][debug] typing-extensions: 4.8.0
[cli][debug] urllib3: 2.1.0
[cli][debug] websocket-client: 1.6.4
[cli][debug] Arguments:
[cli][debug] url=https://piczel.tv/watch/Feretta
[cli][debug] --loglevel=debug
[cli][debug] --player=mpv
[cli][info] Found matching plugin piczel for URL https://piczel.tv/watch/Feretta
[utils.l10n][debug] Language code: en_US
[stream.ffmpegmux][debug] ffmpeg version n6.1 Copyright (c) 2000-2023 the FFmpeg developers
[stream.ffmpegmux][debug] built with gcc 13.2.1 (GCC) 20230801
[stream.ffmpegmux][debug] configuration: --prefix=/usr --disable-debug --disable-static --disable-stripping --enable-amf --enable-avisynth --enable-cuda-llvm --enable-lto --enable-fontconfig --enable-gmp --enable-gnutls --enable-gpl --enable-ladspa --enable-libaom --enable-libass --enable-libbluray --enable-libbs2b --enable-libdav1d --enable-libdrm --enable-libfreetype --enable-libfribidi --enable-libgsm --enable-libiec61883 --enable-libjack --enable-libjxl --enable-libmodplug --enable-libmp3lame --enable-libopencore_amrnb --enable-libopencore_amrwb --enable-libopenjpeg --enable-libopenmpt --enable-libopus --enable-libpulse --enable-librav1e --enable-librsvg --enable-libsoxr --enable-libspeex --enable-libsrt --enable-libssh --enable-libsvtav1 --enable-libtheora --enable-libv4l2 --enable-libvidstab --enable-libvmaf --enable-libvorbis --enable-libvpl --enable-libvpx --enable-libwebp --enable-libx264 --enable-libx265 --enable-libxcb --enable-libxml2 --enable-libxvid --enable-libzimg --enable-nvdec --enable-nvenc --enable-opencl --enable-opengl --enable-shared --enable-version3 --enable-vulkan
[stream.ffmpegmux][debug] libavutil 58. 29.100 / 58. 29.100
[stream.ffmpegmux][debug] libavcodec 60. 31.102 / 60. 31.102
[stream.ffmpegmux][debug] libavformat 60. 16.100 / 60. 16.100
[stream.ffmpegmux][debug] libavdevice 60. 3.100 / 60. 3.100
[stream.ffmpegmux][debug] libavfilter 9. 12.100 / 9. 12.100
[stream.ffmpegmux][debug] libswscale 7. 5.100 / 7. 5.100
[stream.ffmpegmux][debug] libswresample 4. 12.100 / 4. 12.100
[stream.ffmpegmux][debug] libpostproc 57. 3.100 / 57. 3.100
[stream.hls][debug] Using external audio tracks for stream 1080p (language=None, name=Audio_1)
Available streams: 1080p (worst, best)
```
However, there appears to be a muxing issue with their HLS streams:
```
[cli][info] Opening stream: 1080p (hls-multi)
[cli][info] Starting player: mpv
[stream.ffmpegmux][debug] Opening hls substream
[stream.hls][debug] Reloading playlist
[stream.ffmpegmux][debug] Opening hls substream
[stream.hls][debug] Reloading playlist
[utils.named_pipe][info] Creating pipe streamlinkpipe-97906-1-1996
[utils.named_pipe][info] Creating pipe streamlinkpipe-97906-2-3415
[stream.ffmpegmux][debug] ffmpeg command: /usr/bin/ffmpeg -nostats -y -i /tmp/streamlinkpipe-97906-1-1996 -i /tmp/streamlinkpipe-97906-2-3415 -c:v copy -c:a copy -map 0:v? -map 0:a? -map 1:a -f mpegts pipe:1
[stream.ffmpegmux][debug] Starting copy to pipe: /tmp/streamlinkpipe-97906-1-1996
[stream.ffmpegmux][debug] Starting copy to pipe: /tmp/streamlinkpipe-97906-2-3415
[cli][debug] Pre-buffering 8192 bytes
[stream.hls][debug] First Sequence: 0; Last Sequence: 1617
[stream.hls][debug] Start offset: 0; Duration: None; Start Sequence: 1615; End Sequence: None
[stream.hls][debug] Adding segment 1615 to queue
[stream.hls][debug] Adding segment 1616 to queue
[stream.hls][debug] Adding segment 1617 to queue
[stream.hls][debug] Writing segment 1615 to output
[stream.hls][debug] Segment initialization 1615 complete
[stream.hls][debug] First Sequence: 0; Last Sequence: 1617
[stream.hls][debug] Start offset: 0; Duration: None; Start Sequence: 1615; End Sequence: None
[stream.hls][debug] Adding segment 1615 to queue
[stream.hls][debug] Adding segment 1616 to queue
[stream.hls][debug] Adding segment 1617 to queue
[stream.hls][debug] Writing segment 1615 to output
[stream.hls][debug] Segment initialization 1615 complete
[stream.hls][debug] Writing segment 1615 to output
[stream.hls][debug] Segment 1615 complete
[stream.hls][debug] Writing segment 1616 to output
[stream.hls][debug] Segment initialization 1616 complete
[stream.hls][debug] Writing segment 1615 to output
[stream.hls][debug] Segment 1615 complete
[stream.hls][debug] Writing segment 1616 to output
[stream.hls][debug] Segment initialization 1616 complete
[cli.output][debug] Opening subprocess: ['/home/basti/.local/bin/mpv', '--force-media-title=https://piczel.tv/watch/Feretta', '-']
[stream.hls][debug] Writing segment 1616 to output
[stream.hls][debug] Segment 1616 complete
[stream.hls][debug] Writing segment 1617 to output
[stream.hls][debug] Segment initialization 1617 complete
[stream.hls][debug] Writing segment 1617 to output
[stream.hls][debug] Segment 1617 complete
[stream.hls][debug] Writing segment 1616 to output
[stream.hls][debug] Segment 1616 complete
[stream.hls][debug] Writing segment 1617 to output
[stream.hls][debug] Segment initialization 1617 complete
[stream.hls][debug] Writing segment 1617 to output
[stream.hls][debug] Segment 1617 complete
[cli][debug] Writing stream to output
[stream.ffmpegmux][error] Error while writing to pipe /tmp/streamlinkpipe-97906-1-1996: [Errno 32] Broken pipe
[stream.ffmpegmux][error] Error while writing to pipe /tmp/streamlinkpipe-97906-2-3415: [Errno 32] Broken pipe
[stream.ffmpegmux][debug] Closing ffmpeg thread
[stream.segmented][debug] Closing worker thread
[stream.segmented][debug] Closing writer thread
[stream.segmented][debug] Closing worker thread
[stream.segmented][debug] Closing writer thread
[stream.ffmpegmux][debug] Closed all the substreams
[cli][info] Stream ended
[cli][info] Closing currently open stream...
```
Just had a quick look at this again...
After checking FFmpeg's error output using `--ffmpeg-verbose` it looks like it's having issues with Streamlink's output when applying HLS segment maps, aka initialization sections. Those are currently written to the output on each new segment, which is technically wrong, at least for fragmented mp4 segments (still not sure about MPEG-TS segments, but it's very likely the case here as well). Players like VLC and MPV simply skip those duplicate init sections in the output stream, so the current implementation has been working fine in regular unmuxed output streams, but FFmpeg stops remuxing both video and audio streams, so only the first segment works. See #4310.
If I apply a dirty hack that prevents duplicate init sections, then the muxing and final stream output works just fine.
I was in the process of rewriting the segment map logic. See my comment in #5601. This is now a requirement for the plugin to work again. Rewriting this however requires re-organizing the `HLSSegment`, `HLSStreamWorker` and `HLSStreamWriter` classes, because init sections need to be cleared on discontinuities. | 2023-11-26T23:19:34 |
|
streamlink/streamlink | 5,693 | streamlink__streamlink-5693 | [
"5692"
] | f4e12c16c4ae673c4fcddbaebfc672d27fa933a3 | diff --git a/src/streamlink/plugins/bilibili.py b/src/streamlink/plugins/bilibili.py
--- a/src/streamlink/plugins/bilibili.py
+++ b/src/streamlink/plugins/bilibili.py
@@ -4,94 +4,104 @@
$type live
"""
-import logging
import re
-from urllib.parse import urlparse
from streamlink.plugin import Plugin, pluginmatcher
from streamlink.plugin.api import validate
-from streamlink.stream.http import HTTPStream
-
-
-log = logging.getLogger(__name__)
-
-API_URL = "https://api.live.bilibili.com/room/v1/Room/playUrl"
-ROOM_API = "https://api.live.bilibili.com/room/v1/Room/room_init?id={}"
-SHOW_STATUS_OFFLINE = 0
-SHOW_STATUS_ONLINE = 1
-SHOW_STATUS_ROUND = 2
-STREAM_WEIGHTS = {
- "source": 1080,
-}
-
-_room_id_schema = validate.Schema(
- {
- "data": validate.any(None, {
- "room_id": int,
- "live_status": int,
- }),
- },
- validate.get("data"),
-)
-
-_room_stream_list_schema = validate.Schema(
- {
- "data": validate.any(None, {
- "durl": [{"url": validate.url()}],
- }),
- },
- validate.get("data"),
-)
+from streamlink.stream.hls import HLSStream
@pluginmatcher(re.compile(
r"https?://live\.bilibili\.com/(?P<channel>[^/]+)",
))
class Bilibili(Plugin):
- @classmethod
- def stream_weight(cls, stream):
- if stream in STREAM_WEIGHTS:
- return STREAM_WEIGHTS[stream], "Bilibili"
-
- return Plugin.stream_weight(stream)
+ SHOW_STATUS_OFFLINE = 0
+ SHOW_STATUS_ONLINE = 1
+ SHOW_STATUS_ROUND = 2
def _get_streams(self):
- self.session.http.headers.update({"Referer": self.url})
- channel = self.match.group("channel")
- res_room_id = self.session.http.get(ROOM_API.format(channel))
- room_id_json = self.session.http.json(res_room_id, schema=_room_id_schema)
- room_id = room_id_json["room_id"]
- if room_id_json["live_status"] != SHOW_STATUS_ONLINE:
+ schema_stream = validate.all(
+ [{
+ "protocol_name": str,
+ "format": validate.all(
+ [{
+ "format_name": str,
+ "codec": validate.all(
+ [{
+ "codec_name": str,
+ "base_url": str,
+ "url_info": [{
+ "host": validate.url(),
+ "extra": str,
+ }],
+ }],
+ validate.filter(lambda item: item["codec_name"] == "avc"),
+ ),
+ }],
+ validate.filter(lambda item: item["format_name"] == "fmp4"),
+ ),
+ }],
+ validate.filter(lambda item: item["protocol_name"] == "http_hls"),
+ )
+
+ data = self.session.http.get(
+ self.url,
+ schema=validate.Schema(
+ validate.parse_html(),
+ validate.xml_xpath_string(".//script[contains(text(),'window.__NEPTUNE_IS_MY_WAIFU__={')][1]/text()"),
+ validate.none_or_all(
+ validate.transform(str.replace, "window.__NEPTUNE_IS_MY_WAIFU__=", ""),
+ validate.parse_json(),
+ {
+ "roomInitRes": {
+ "data": {
+ "live_status": int,
+ "playurl_info": {
+ "playurl": {
+ "stream": schema_stream,
+ },
+ },
+ },
+ },
+ "roomInfoRes": {
+ "data": {
+ "room_info": {
+ "live_id": int,
+ "title": str,
+ "area_name": str,
+ },
+ "anchor_info": {
+ "base_info": {
+ "uname": str,
+ },
+ },
+ },
+ },
+ },
+ validate.union_get(
+ ("roomInfoRes", "data", "room_info", "live_id"),
+ ("roomInfoRes", "data", "anchor_info", "base_info", "uname"),
+ ("roomInfoRes", "data", "room_info", "area_name"),
+ ("roomInfoRes", "data", "room_info", "title"),
+ ("roomInitRes", "data", "live_status"),
+ ("roomInitRes", "data", "playurl_info", "playurl", "stream"),
+ ),
+ ),
+ ),
+ )
+ if not data:
return
- params = {
- "cid": room_id,
- "quality": "4",
- "platform": "web",
- }
- res = self.session.http.get(API_URL, params=params)
- room = self.session.http.json(res, schema=_room_stream_list_schema)
- if not room:
+ self.id, self.author, self.category, self.title, live_status, streams = data
+ if live_status != self.SHOW_STATUS_ONLINE:
return
- for stream_list in room["durl"]:
- name = "source"
- url = stream_list["url"]
- # check if the URL is available
- log.trace("URL={0}".format(url))
- r = self.session.http.get(url,
- retries=0,
- timeout=3,
- stream=True,
- acceptable_status=(200, 403, 404, 405))
- p = urlparse(url)
- if r.status_code != 200:
- log.error("Netloc: {0} with error {1}".format(p.netloc, r.status_code))
- continue
-
- log.debug("Netloc: {0}".format(p.netloc))
- stream = HTTPStream(self.session, url)
- yield name, stream
+ for stream in streams:
+ for stream_format in stream["format"]:
+ for codec in stream_format["codec"]:
+ for url_info in codec["url_info"]:
+ url = f"{url_info['host']}{codec['base_url']}{url_info['extra']}"
+ yield "live", HLSStream(self.session, url)
__plugin__ = Bilibili
| plugins.bilibili: Some live streams cannot be obtained
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
6.4.1
### Description
Some live broadcast browsers on station B can be watched, but cannot be obtained using streamlink. Some even have a black screen when viewed through browsers. I guess it may be a CDN problem.
### Debug log
```text
streamlink https://live.bilibili.com/24022841 best --loglevel=debug
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.11.6
[cli][debug] OpenSSL: OpenSSL 3.0.11 19 Sep 2023
[cli][debug] Streamlink: 6.4.1
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.11.17
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.3
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.19.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.23.1
[cli][debug] trio-websocket: 0.11.1
[cli][debug] typing-extensions: 4.8.0
[cli][debug] urllib3: 2.1.0
[cli][debug] websocket-client: 1.6.4
[cli][debug] Arguments:
[cli][debug] url=https://live.bilibili.com/24022841
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][info] Found matching plugin bilibili for URL https://live.bilibili.com/24022841
error: Unable to validate JSON: ValidationError(dict):
Unable to validate value of key 'data'
Context(AnySchema):
ValidationError(equality):
<{'current_quality': 0, 'accept_quality': None, 'current...> does not equal None
ValidationError(dict):
Key 'durl' not found in <{'current_quality': 0, 'accept_quality': None, 'current...>
```
| The plugin requires a full rewrite...
The stream URLs are now embedded as JSON on the live URL itself. I have already a working re-implementation of the plugin in my local git repo. However, they only appear to have low-quality streams for regular users. The streamer's input stream and re-encoded high-quality streams all require a login, and creating a new account requires a phone number or some Chinese social media accounts, so this is a dead end for me. | 2023-11-28T14:56:58 |
|
streamlink/streamlink | 5,698 | streamlink__streamlink-5698 | [
"5697"
] | 7e722ec1219ce3886a0af1c43b4fbf5ba3aad51d | diff --git a/src/streamlink/plugins/btv.py b/src/streamlink/plugins/btv.py
--- a/src/streamlink/plugins/btv.py
+++ b/src/streamlink/plugins/btv.py
@@ -61,7 +61,7 @@ def _get_streams(self):
log.error("The content is not available in your region")
return
- return {"live": HLSStream(self.session, stream_url)}
+ return HLSStream.parse_variant_playlist(self.session, stream_url)
__plugin__ = BTV
| diff --git a/tests/plugins/test_btv.py b/tests/plugins/test_btv.py
--- a/tests/plugins/test_btv.py
+++ b/tests/plugins/test_btv.py
@@ -6,7 +6,7 @@ class TestPluginCanHandleUrlBTV(PluginCanHandleUrl):
__plugin__ = BTV
should_match = [
- "http://btvplus.bg/live",
- "http://btvplus.bg/live/",
- "http://www.btvplus.bg/live/",
+ "https://btvplus.bg/live",
+ "https://btvplus.bg/live/",
+ "https://www.btvplus.bg/live/",
]
| plugins.btv: No playable streams found
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Your Streamlink version (6.4.2+1.g7e722ec1) is up to date!
### Description
The plug-in does not display video. It displays errors shown in the logs below.
### Debug log
```text
streamlink --loglevel=debug "https://btvplus.bg/live/" best
[cli][debug] OS: Linux-6.2.0-35-generic-x86_64-with-glibc2.35
[cli][debug] Python: 3.10.12
[cli][debug] OpenSSL: OpenSSL 3.0.2 15 Mar 2022
[cli][debug] Streamlink: 6.4.2+1.g7e722ec1
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.5.7
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.8.0
[cli][debug] pycountry: 20.7.3
[cli][debug] pycryptodome: 3.17
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.22.2
[cli][debug] trio-websocket: 0.10.3
[cli][debug] typing-extensions: 4.7.1
[cli][debug] urllib3: 1.26.16
[cli][debug] websocket-client: 1.2.3
[cli][debug] Arguments:
[cli][debug] url=https://btvplus.bg/live/
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][info] Found matching plugin btv for URL https://btvplus.bg/live/
[cli][info] Available streams: live (worst, best)
[cli][info] Opening stream: live (hls)
[cli][info] Starting player: /usr/bin/vlc
[stream.hls][debug] Reloading playlist
[cli][debug] Pre-buffering 8192 bytes
[stream.hls][error] Attempted to play a variant playlist, use 'hls://https://cdn.bweb.bg/live/PhRBlmfjy0uVGxaj1_BMiw/1701627017/61065646.m3u8' instead
[stream.segmented][debug] Closing worker thread
[stream.segmented][debug] Closing writer thread
[cli][error] Try 1/1: Could not open stream <HLSStream ['hls', 'https://cdn.bweb.bg/live/PhRBlmfjy0uVGxaj1_BMiw/1701627017/61065646.m3u8']> (No data returned from stream)
error: Could not open stream <HLSStream ['hls', 'https://cdn.bweb.bg/live/PhRBlmfjy0uVGxaj1_BMiw/1701627017/61065646.m3u8']>, tried 1 times, exiting
[cli][info] Closing currently open stream...
```
| 2023-12-03T11:49:54 |
|
streamlink/streamlink | 5,704 | streamlink__streamlink-5704 | [
"5703",
"5656"
] | 2d751a530b34dc1c4b8710acb76f614fe2145a08 | diff --git a/src/streamlink/plugins/twitch.py b/src/streamlink/plugins/twitch.py
--- a/src/streamlink/plugins/twitch.py
+++ b/src/streamlink/plugins/twitch.py
@@ -495,8 +495,16 @@ def stream_metadata(self, channel):
)
return self.call(query, schema=validate.Schema(
- {"data": {"user": {"stream": {"type": str}}}},
- validate.get(("data", "user", "stream")),
+ {
+ "data": {
+ "user": validate.none_or_all({
+ "stream": validate.none_or_all({
+ "id": str,
+ }),
+ }),
+ },
+ },
+ validate.get(("data", "user")),
))
@@ -640,7 +648,7 @@ def decode_client_integrity_token(data: str, schema: Optional[validate.Schema] =
@pluginargument(
"disable-reruns",
action="store_true",
- help="Do not open the stream if the target channel is currently broadcasting a rerun.",
+ help=argparse.SUPPRESS,
)
@pluginargument(
"low-latency",
@@ -808,22 +816,19 @@ def _access_token(self, is_live, channel_or_vod):
return sig, token, restricted_bitrates
- def _check_for_rerun(self):
- if not self.options.get("disable_reruns"):
+ def _check_is_live(self) -> bool:
+ data = self.api.stream_metadata(self.channel)
+ if data is None:
+ log.error("Unknown channel")
+ return False
+ if data["stream"] is None:
+ log.error("Channel is offline")
return False
- try:
- stream = self.api.stream_metadata(self.channel)
- if stream["type"] != "live":
- log.info("Reruns were disabled by command line option")
- return True
- except (PluginError, TypeError):
- pass
-
- return False
+ return True
def _get_hls_streams_live(self):
- if self._check_for_rerun():
+ if not self._check_is_live():
return
# only get the token once the channel has been resolved
| diff --git a/tests/plugins/test_twitch.py b/tests/plugins/test_twitch.py
--- a/tests/plugins/test_twitch.py
+++ b/tests/plugins/test_twitch.py
@@ -396,6 +396,53 @@ def test_hls_low_latency_no_ads_reload_time(self):
assert self.thread.reader.worker.playlist_reload_time == pytest.approx(23 / 3)
+class TestTwitchIsLive:
+ @pytest.fixture()
+ def plugin(self, request: pytest.FixtureRequest):
+ return Twitch(Streamlink(), "https://twitch.tv/channelname")
+
+ @pytest.fixture()
+ def mock(self, request: pytest.FixtureRequest, requests_mock: rm.Mocker):
+ mock = requests_mock.post("https://gql.twitch.tv/gql", **getattr(request, "param", {"json": {}}))
+ yield mock
+ assert mock.call_count > 0
+ payload = mock.last_request.json() # type: ignore[union-attr]
+ assert tuple(sorted(payload.keys())) == ("extensions", "operationName", "variables")
+ assert payload.get("operationName") == "StreamMetadata"
+ assert payload.get("extensions") == {
+ "persistedQuery": {
+ "sha256Hash": "1c719a40e481453e5c48d9bb585d971b8b372f8ebb105b17076722264dfa5b3e",
+ "version": 1,
+ },
+ }
+ assert payload.get("variables") == {"channelLogin": "channelname"}
+
+ @pytest.mark.parametrize(("mock", "expected", "log"), [
+ pytest.param(
+ {"json": {"data": {"user": None}}},
+ False,
+ [("streamlink.plugins.twitch", "error", "Unknown channel")],
+ id="no-user",
+ ),
+ pytest.param(
+ {"json": {"data": {"user": {"stream": None}}}},
+ False,
+ [("streamlink.plugins.twitch", "error", "Channel is offline")],
+ id="no-stream",
+ ),
+ pytest.param(
+ {"json": {"data": {"user": {"stream": {"id": "1234567890"}}}}},
+ True,
+ [],
+ id="is-live",
+ ),
+ ], indirect=["mock"])
+ def test_is_live(self, plugin: Twitch, caplog: pytest.LogCaptureFixture, mock: rm.Mocker, expected: bool, log: list):
+ caplog.set_level(1, "streamlink")
+ assert plugin._check_is_live() is expected
+ assert [(record.name, record.levelname, record.message) for record in caplog.records] == log
+
+
class TestTwitchAPIAccessToken:
@pytest.fixture(autouse=True)
def _client_integrity_token(self, monkeypatch: pytest.MonkeyPatch):
@@ -834,63 +881,3 @@ def test_metadata_clip_no_data(self, mock_request_clip, metadata):
assert author is None
assert category is None
assert title is None
-
-
[email protected](("stream_type", "offline", "disable", "expected", "logs"), [
- pytest.param(
- "live",
- False,
- True,
- False,
- [],
- id="disable live",
- ),
- pytest.param(
- "rerun",
- False,
- True,
- True,
- [("streamlink.plugins.twitch", "info", "Reruns were disabled by command line option")],
- id="disable not live",
- ),
- pytest.param(
- "live",
- True,
- True,
- False,
- [],
- id="disable offline",
- ),
- pytest.param(
- "rerun",
- True,
- False,
- False,
- [],
- id="enable",
- ),
-])
-def test_reruns(
- monkeypatch: pytest.MonkeyPatch,
- caplog: pytest.LogCaptureFixture,
- session: Streamlink,
- stream_type: str,
- offline: bool,
- disable: bool,
- expected: bool,
- logs: list,
-):
- caplog.set_level(1, "streamlink")
- mock_stream_metadata = Mock(return_value=None if offline else {"type": stream_type})
- monkeypatch.setattr("streamlink.plugins.twitch.TwitchAPI.stream_metadata", mock_stream_metadata)
-
- # noinspection PyTypeChecker
- plugin: Twitch = Twitch(session, "https://www.twitch.tv/foo")
- try:
- plugin.options.set("disable-reruns", disable)
- result = plugin._check_for_rerun()
- finally:
- plugin.options.clear()
-
- assert result is expected
- assert [(record.name, record.levelname, record.message) for record in caplog.records] == logs
| plugins.twitch: returns streams while channel is offline
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
streamlink 6.4.2
### Description
The --retry-streams switch seems to be broken on twitch.
I keep running `streamlink --retry-streams X` so I can record VODs of my favorite streamer. Last night, after their stream ended, streamlink kept exiting instead of retrying to record a stream.
Steps to reproduce:
`streamlink twitch.tv/any-offline-streamer best -o "/disk1/share1/twitch/{time}.ts" --retry-streams 5`
Expected behavior: streamlink detects no live stream and keeps on trying to record every 5 seconds.
What actually happens:
```
[stream.hls][error] Unable to open URL: https://video-weaver.fra02.hls.ttvnw.net/v1/playlist/Ct8E[...]
[cli][error] Try 1/1: Could not open stream <TwitchHLSStream ['hls', 'https://video-weaver.fra02.hls.ttvnw.net/v1/playlist/Ct8E[...]
(No data returned from stream)
error: Could not open stream <TwitchHLSStream ['hls', 'https://video-weaver.fra02.hls.ttvnw.net/v1/playlist/Ct8E
[...] tried 1 times, exiting
```
### Debug log
```text
[cli][debug] OS: Linux-5.10.0-26-amd64-x86_64-with-glibc2.31
[cli][debug] Python: 3.9.2
[cli][debug] OpenSSL: OpenSSL 1.1.1w 11 Sep 2023
[cli][debug] Streamlink: 6.4.2
[cli][debug] Dependencies:
[cli][debug] certifi: 2020.6.20
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.1
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.16.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.22.2
[cli][debug] trio-websocket: 0.10.3
[cli][debug] typing-extensions: 4.7.1
[cli][debug] urllib3: 2.1.0
[cli][debug] websocket-client: 1.4.2
[cli][debug] Arguments:
[cli][debug] url=twitch.tv/streamername
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --output=/disk1/share1/twitch/{time}.ts
[cli][debug] --retry-streams=5.0
[cli][debug] --twitch-api-header=[('Authorization', 'OAuth oauthkey')]
[cli][info] Found matching plugin twitch for URL twitch.tv/streamername
[plugins.twitch][debug] Getting live HLS streams for streamername
[plugins.twitch][debug] {'adblock': False, 'geoblock_reason': '', 'hide_ads': False, 'server_ads': True, 'show_ads': True}
[utils.l10n][debug] Language code: en_US
[cli][info] Available streams: audio_only, 160p (worst), 360p, 480p, 720p60, 1080p60 (best)
[cli][info] Opening stream: 1080p60 (hls)
[cli][info] Writing output to
/disk1/share1/twitch/2023-12-05_06-50-04.ts
[cli][debug] Checking file output
[stream.hls][debug] Reloading playlist
[cli][debug] Pre-buffering 8192 bytes
[stream.hls][error] Unable to open URL: https://video-weaver.fra02.hls.ttvnw.net/v1/playlist/Ct8Et7-cohsd9159aO2PPQ6Seos4y4HOvcb72tOtDdRNTj8hpKZlbvCMgSrL0JzJEezXEL7893RUjYW-NTfbZcZOXn19u5IrlV9sBJBFYrZp2tL0VAszcZNOI2TN-hBp3vdd-H2H19i6EcZUEMGjDjt_pdgVd9WX9OTQqPRqmK9I1GN8bOQ7yKXjQiwQPj_5puEfGNPAP-IIqZ6FsMlGykkectRfLsSQ0Cg4W5IkiHnoEVeY6DbalTBUZHoAlmENk6p-sO1iRCunKdFWZ7U9ac0uJn8yBybSTNUwYzV-uYJZuvbbZQxrGHjjCqRXzi_NDGNQQKwm0-vd1QUdSEKdBKwcqgpm-Dv8IZGupIYXwQZOS0nUesayUc0ENP4ELbMn-_R61RD9xJU_xnQq-0BjLuMvzscu4YG74LHXs5O2N114OdrX2nK--GqmF-QDoY3z28F2QTS1QmwKgjFeO0W2zxAczEEJcR-f-_VKZCumbNDwWPI7TViy79nyYAnC64377Js0m7bFO4wn-10y9WvokvkPhpxkgTlUwDJCKrXnGmcnefr1QNQ6U27U0Un3S6heB8KUL_STsDi3zZ7gYx58vCcvFU0v5LsNE2eBjCQnHA7Z0OQQBQXAo1nJKtG1f8xNAQQyXr8gJVZSTjn29a0BGT-AmhF3MpJIHKRV1kFCQOeA8jz8nU6eUms6eOWRrh1uvX1O-IjHNy82zvBA0t8cpxaGtnS_SVH1E81RcbG0YpPtH4lcs1i7yzQpIqPg1eLdvUjrVbPaIvRiaL-VIjLNPIKQ21V-hyoe5ALJzwT5I904WhoM5VDKLPcrcqh3JdE7IAEqCXVzLXdlc3QtMjCUCA.m3u8 (404 Client Error: Not Found for url: https://video-weaver.fra02.hls.ttvnw.net/v1/playlist/Ct8Et7-cohsd9159aO2PPQ6Seos4y4HOvcb72tOtDdRNTj8hpKZlbvCMgSrL0JzJEezXEL7893RUjYW-NTfbZcZOXn19u5IrlV9sBJBFYrZp2tL0VAszcZNOI2TN-hBp3vdd-H2H19i6EcZUEMGjDjt_pdgVd9WX9OTQqPRqmK9I1GN8bOQ7yKXjQiwQPj_5puEfGNPAP-IIqZ6FsMlGykkectRfLsSQ0Cg4W5IkiHnoEVeY6DbalTBUZHoAlmENk6p-sO1iRCunKdFWZ7U9ac0uJn8yBybSTNUwYzV-uYJZuvbbZQxrGHjjCqRXzi_NDGNQQKwm0-vd1QUdSEKdBKwcqgpm-Dv8IZGupIYXwQZOS0nUesayUc0ENP4ELbMn-_R61RD9xJU_xnQq-0BjLuMvzscu4YG74LHXs5O2N114OdrX2nK--GqmF-QDoY3z28F2QTS1QmwKgjFeO0W2zxAczEEJcR-f-_VKZCumbNDwWPI7TViy79nyYAnC64377Js0m7bFO4wn-10y9WvokvkPhpxkgTlUwDJCKrXnGmcnefr1QNQ6U27U0Un3S6heB8KUL_STsDi3zZ7gYx58vCcvFU0v5LsNE2eBjCQnHA7Z0OQQBQXAo1nJKtG1f8xNAQQyXr8gJVZSTjn29a0BGT-AmhF3MpJIHKRV1kFCQOeA8jz8nU6eUms6eOWRrh1uvX1O-IjHNy82zvBA0t8cpxaGtnS_SVH1E81RcbG0YpPtH4lcs1i7yzQpIqPg1eLdvUjrVbPaIvRiaL-VIjLNPIKQ21V-hyoe5ALJzwT5I904WhoM5VDKLPcrcqh3JdE7IAEqCXVzLXdlc3QtMjCUCA.m3u8)
[stream.segmented][debug] Closing worker thread
[stream.segmented][debug] Closing writer thread
[cli][error] Try 1/1: Could not open stream <TwitchHLSStream ['hls', 'https://video-weaver.fra02.hls.ttvnw.net/v1/playlist/Ct8Et7-cohsd9159aO2PPQ6Seos4y4HOvcb72tOtDdRNTj8hpKZlbvCMgSrL0JzJEezXEL7893RUjYW-NTfbZcZOXn19u5IrlV9sBJBFYrZp2tL0VAszcZNOI2TN-hBp3vdd-H2H19i6EcZUEMGjDjt_pdgVd9WX9OTQqPRqmK9I1GN8bOQ7yKXjQiwQPj_5puEfGNPAP-IIqZ6FsMlGykkectRfLsSQ0Cg4W5IkiHnoEVeY6DbalTBUZHoAlmENk6p-sO1iRCunKdFWZ7U9ac0uJn8yBybSTNUwYzV-uYJZuvbbZQxrGHjjCqRXzi_NDGNQQKwm0-vd1QUdSEKdBKwcqgpm-Dv8IZGupIYXwQZOS0nUesayUc0ENP4ELbMn-_R61RD9xJU_xnQq-0BjLuMvzscu4YG74LHXs5O2N114OdrX2nK--GqmF-QDoY3z28F2QTS1QmwKgjFeO0W2zxAczEEJcR-f-_VKZCumbNDwWPI7TViy79nyYAnC64377Js0m7bFO4wn-10y9WvokvkPhpxkgTlUwDJCKrXnGmcnefr1QNQ6U27U0Un3S6heB8KUL_STsDi3zZ7gYx58vCcvFU0v5LsNE2eBjCQnHA7Z0OQQBQXAo1nJKtG1f8xNAQQyXr8gJVZSTjn29a0BGT-AmhF3MpJIHKRV1kFCQOeA8jz8nU6eUms6eOWRrh1uvX1O-IjHNy82zvBA0t8cpxaGtnS_SVH1E81RcbG0YpPtH4lcs1i7yzQpIqPg1eLdvUjrVbPaIvRiaL-VIjLNPIKQ21V-hyoe5ALJzwT5I904WhoM5VDKLPcrcqh3JdE7IAEqCXVzLXdlc3QtMjCUCA.m3u8', 'https://usher.ttvnw.net/api/channel/hls/streamername.m3u8?player=twitchweb&p=17828&type=any&allow_source=true&allow_audio_only=true&allow_spectre=false&sig=e99f77d8cdd6aa3055787fddba62db0d8dddc3d6&token=%7B%22adblock%22%3Afalse%2C%22authorization%22%3A%7B%22forbidden%22%3Afalse%2C%22reason%22%3A%22%22%7D%2C%22blackout_enabled%22%3Afalse%2C%22channel%22%3A%22streamername%22%2C%22channel_id%22%3A38251312%2C%22chansub%22%3A%7B%22restricted_bitrates%22%3A%5B%5D%2C%22view_until%22%3A1924905600%7D%2C%22ci_gb%22%3Afalse%2C%22geoblock_reason%22%3A%22%22%2C%22device_id%22%3Anull%2C%22expires%22%3A1701756604%2C%22extended_history_allowed%22%3Afalse%2C%22game%22%3A%22%22%2C%22hide_ads%22%3Afalse%2C%22https_required%22%3Atrue%2C%22mature%22%3Afalse%2C%22partner%22%3Afalse%2C%22platform%22%3A%22web%22%2C%22player_type%22%3A%22embed%22%2C%22private%22%3A%7B%22allowed_to_view%22%3Atrue%7D%2C%22privileged%22%3Afalse%2C%22role%22%3A%22%22%2C%22server_ads%22%3Atrue%2C%22show_ads%22%3Atrue%2C%22subscriber%22%3Afalse%2C%22turbo%22%3Afalse%2C%22user_id%22%3A495478723%2C%22user_ip%22%3A%2285.67.13.26%22%2C%22version%22%3A2%7D&fast_bread=True']> (No data returned from stream)
error: Could not open stream <TwitchHLSStream ['hls', 'https://video-weaver.fra02.hls.ttvnw.net/v1/playlist/Ct8Et7-cohsd9159aO2PPQ6Seos4y4HOvcb72tOtDdRNTj8hpKZlbvCMgSrL0JzJEezXEL7893RUjYW-NTfbZcZOXn19u5IrlV9sBJBFYrZp2tL0VAszcZNOI2TN-hBp3vdd-H2H19i6EcZUEMGjDjt_pdgVd9WX9OTQqPRqmK9I1GN8bOQ7yKXjQiwQPj_5puEfGNPAP-IIqZ6FsMlGykkectRfLsSQ0Cg4W5IkiHnoEVeY6DbalTBUZHoAlmENk6p-sO1iRCunKdFWZ7U9ac0uJn8yBybSTNUwYzV-uYJZuvbbZQxrGHjjCqRXzi_NDGNQQKwm0-vd1QUdSEKdBKwcqgpm-Dv8IZGupIYXwQZOS0nUesayUc0ENP4ELbMn-_R61RD9xJU_xnQq-0BjLuMvzscu4YG74LHXs5O2N114OdrX2nK--GqmF-QDoY3z28F2QTS1QmwKgjFeO0W2zxAczEEJcR-f-_VKZCumbNDwWPI7TViy79nyYAnC64377Js0m7bFO4wn-10y9WvokvkPhpxkgTlUwDJCKrXnGmcnefr1QNQ6U27U0Un3S6heB8KUL_STsDi3zZ7gYx58vCcvFU0v5LsNE2eBjCQnHA7Z0OQQBQXAo1nJKtG1f8xNAQQyXr8gJVZSTjn29a0BGT-AmhF3MpJIHKRV1kFCQOeA8jz8nU6eUms6eOWRrh1uvX1O-IjHNy82zvBA0t8cpxaGtnS_SVH1E81RcbG0YpPtH4lcs1i7yzQpIqPg1eLdvUjrVbPaIvRiaL-VIjLNPIKQ21V-hyoe5ALJzwT5I904WhoM5VDKLPcrcqh3JdE7IAEqCXVzLXdlc3QtMjCUCA.m3u8', 'https://usher.ttvnw.net/api/channel/hls/streamername.m3u8?player=twitchweb&p=17828&type=any&allow_source=true&allow_audio_only=true&allow_spectre=false&sig=e99f77d8cdd6aa3055787fddba62db0d8dddc3d6&token=%7B%22adblock%22%3Afalse%2C%22authorization%22%3A%7B%22forbidden%22%3Afalse%2C%22reason%22%3A%22%22%7D%2C%22blackout_enabled%22%3Afalse%2C%22channel%22%3A%22streamername%22%2C%22channel_id%22%3A38251312%2C%22chansub%22%3A%7B%22restricted_bitrates%22%3A%5B%5D%2C%22view_until%22%3A1924905600%7D%2C%22ci_gb%22%3Afalse%2C%22geoblock_reason%22%3A%22%22%2C%22device_id%22%3Anull%2C%22expires%22%3A1701756604%2C%22extended_history_allowed%22%3Afalse%2C%22game%22%3A%22%22%2C%22hide_ads%22%3Afalse%2C%22https_required%22%3Atrue%2C%22mature%22%3Afalse%2C%22partner%22%3Afalse%2C%22platform%22%3A%22web%22%2C%22player_type%22%3A%22embed%22%2C%22private%22%3A%7B%22allowed_to_view%22%3Atrue%7D%2C%22privileged%22%3Afalse%2C%22role%22%3A%22%22%2C%22server_ads%22%3Atrue%2C%22show_ads%22%3Atrue%2C%22subscriber%22%3Afalse%2C%22turbo%22%3Afalse%2C%22user_id%22%3A495478723%2C%22user_ip%22%3A%2285.67.13.26%22%2C%22version%22%3A2%7D&fast_bread=True']>, tried 1 times, exiting
[cli][info] Closing currently open stream...
```
plugins.twitch: latest update started flooding console with confusing messages
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
6.3.1
### Description
did someone enable a new debug setting or something and how to turn that off if so? the plugin appears to work but it has started flooding the console with messages. its not the end of the world but i would like the console clean like it used to be
### Debug log
```text
[plugins.twitch][error] twirp error not_found: transcode does not exist
[plugins.twitch][error] Could not access HLS playlist
```
| `--retry-streams` has nothing to do with any implementation of Streamlink's plugins. It's a feature of `streamlink_cli` which keeps fetching streams from the resolved plugin until ones were found.
As you can see in your debug log output, the plugin has successfully returned a list of streams:
> [cli][info] Available streams: audio_only, 160p (worst), 360p, 480p, 720p60, 1080p60 (best)
The reason why you can't access the streams is that Twitch is returning a 404 HTTP status code when trying to access the HLS media playlist. Since you redacted the channel name, I can't tell you what the issue is. Maybe it's because of your OAuth token, maybe not... You'll have to provide more information.
Actual offline streams are working as intended, as you can see here, as well as in #5656:
```
$ streamlink --no-config -l debug --retry-streams 5 twitch.tv/twitch best
[cli][debug] OS: Linux-6.6.3-1-git-x86_64-with-glibc2.38
[cli][debug] Python: 3.11.6
[cli][debug] OpenSSL: OpenSSL 3.1.4 24 Oct 2023
[cli][debug] Streamlink: 6.4.2+2.g71e885e0
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.11.17
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.3
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.19.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.23.1
[cli][debug] trio-websocket: 0.11.1
[cli][debug] typing-extensions: 4.8.0
[cli][debug] urllib3: 2.1.0
[cli][debug] websocket-client: 1.6.4
[cli][debug] Arguments:
[cli][debug] url=twitch.tv/twitch
[cli][debug] stream=['best']
[cli][debug] --no-config=True
[cli][debug] --loglevel=debug
[cli][debug] --retry-streams=5.0
[cli][info] Found matching plugin twitch for URL twitch.tv/twitch
[plugins.twitch][debug] Getting live HLS streams for twitch
[plugins.twitch][debug] {'adblock': False, 'geoblock_reason': '', 'hide_ads': False, 'server_ads': True, 'show_ads': True}
[utils.l10n][debug] Language code: en_US
[plugins.twitch][error] Could not access HLS playlist
[cli][info] Waiting for streams, retrying every 5.0 second(s)
[plugins.twitch][debug] Getting live HLS streams for twitch
[plugins.twitch][debug] {'adblock': False, 'geoblock_reason': '', 'hide_ads': False, 'server_ads': True, 'show_ads': True}
[utils.l10n][debug] Language code: en_US
[plugins.twitch][error] Could not access HLS playlist
[plugins.twitch][debug] Getting live HLS streams for twitch
[plugins.twitch][debug] {'adblock': False, 'geoblock_reason': '', 'hide_ads': False, 'server_ads': True, 'show_ads': True}
[utils.l10n][debug] Language code: en_US
[plugins.twitch][error] Could not access HLS playlist
^CInterrupted! Exiting...
```
```
$ streamlink --no-config -l debug --retry-streams 5 twitch.tv/lirik best
[cli][debug] OS: Linux-6.6.3-1-git-x86_64-with-glibc2.38
[cli][debug] Python: 3.11.6
[cli][debug] OpenSSL: OpenSSL 3.1.4 24 Oct 2023
[cli][debug] Streamlink: 6.4.2+2.g71e885e0
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.11.17
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.3
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.19.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.23.1
[cli][debug] trio-websocket: 0.11.1
[cli][debug] typing-extensions: 4.8.0
[cli][debug] urllib3: 2.1.0
[cli][debug] websocket-client: 1.6.4
[cli][debug] Arguments:
[cli][debug] url=twitch.tv/lirik
[cli][debug] stream=['best']
[cli][debug] --no-config=True
[cli][debug] --loglevel=debug
[cli][debug] --retry-streams=5.0
[cli][info] Found matching plugin twitch for URL twitch.tv/lirik
[plugins.twitch][debug] Getting live HLS streams for lirik
[plugins.twitch][debug] {'adblock': False, 'geoblock_reason': '', 'hide_ads': False, 'server_ads': True, 'show_ads': True}
[utils.l10n][debug] Language code: en_US
[plugins.twitch][error] twirp error not_found: transcode does not exist
[cli][info] Waiting for streams, retrying every 5.0 second(s)
[plugins.twitch][debug] Getting live HLS streams for lirik
[plugins.twitch][debug] {'adblock': False, 'geoblock_reason': '', 'hide_ads': False, 'server_ads': True, 'show_ads': True}
[utils.l10n][debug] Language code: en_US
[plugins.twitch][error] twirp error not_found: transcode does not exist
[plugins.twitch][debug] Getting live HLS streams for lirik
[plugins.twitch][debug] {'adblock': False, 'geoblock_reason': '', 'hide_ads': False, 'server_ads': True, 'show_ads': True}
[utils.l10n][debug] Language code: en_US
[plugins.twitch][error] twirp error not_found: transcode does not exist
^CInterrupted! Exiting...
```
Thank you for the reply. I did have my OAuth token in the config. Removed it (as in: moved the config file elsewhere as the only line in it was for the token) for testing and it's still the same error. The channel I am trying with is: twitch.tv/paymoneywubby
```
[cli][debug] OS: Linux-5.10.0-26-amd64-x86_64-with-glibc2.31
[cli][debug] Python: 3.9.2
[cli][debug] OpenSSL: OpenSSL 1.1.1w 11 Sep 2023
[cli][debug] Streamlink: 6.4.2
[cli][debug] Dependencies:
[cli][debug] certifi: 2020.6.20
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.1
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.16.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.22.2
[cli][debug] trio-websocket: 0.10.3
[cli][debug] typing-extensions: 4.7.1
[cli][debug] urllib3: 2.1.0
[cli][debug] websocket-client: 1.4.2
[cli][debug] Arguments:
[cli][debug] url=twitch.tv/paymoneywubby
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --output=/disk1/share1/twitch/{time}.ts
[cli][debug] --retry-streams=5.0
[cli][info] Found matching plugin twitch for URL twitch.tv/paymoneywubby
[plugins.twitch][debug] Getting live HLS streams for paymoneywubby
[plugins.twitch][debug] {'adblock': False, 'geoblock_reason': '', 'hide_ads': False, 'server_ads': True, 'show_ads': True}
[utils.l10n][debug] Language code: en_US
[cli][info] Available streams: audio_only, 160p (worst), 360p, 480p, 720p60, 1080p60 (best)
[cli][info] Opening stream: 1080p60 (hls)
[cli][info] Writing output to
/disk1/share1/twitch/2023-12-05_08-00-15.ts
[cli][debug] Checking file output
[stream.hls][debug] Reloading playlist
[cli][debug] Pre-buffering 8192 bytes
[stream.hls][error] Unable to open URL: https://video-weaver.fra02.hls.ttvnw.net/v1/playlist/CtIEZDm7NWyT-thnAUuZBiU69sb15zPPD9XZbKlLNGM8kvad-SgolHdrrncU1szlHTkQv-jjeNG8kWbTjajFkzJqI0vrnDaLh7BleaEqPGenNfXUYngYyPITTncELfAq8sjn0c3yFFFIao7CnoLfbDYC_NqOrDGJ0PAQGQaMnN3xrrIXEFxPOIYaIhtNMsbMZj7q40kVRD8lo4bYdB6IFxD5PQT2WPIJa2ugseh4Rn5NQw-Tf_pRQvhGVxinA_llE02hm-_pF0f2i-2KrXgvOFIqPqYC_7Nxc0OqDTYZsYW-PwFridPkWrT9R1Q7oWzepMFWBoXkhnlHFQGxW1-Efn3HGY5goejUggEFikuw97m4MU5SilCehYv8UbB8sDKsfUFTdwS1fz7Gp4BdcmgjcSj22Xn3oBC4V-JUVpJoV53gc_vTVrjlbHbGnBuF2MBbZz8Tazk53YmWgVU_QE2ZubX1qLCdlbwbRfckm23XADHpuC3sZyxS1CVh76m4ZXA4WGeW2y-LmriJnLj31h1awSpRADjJS0uDFOT8NPPiafZgXaTV6Y9-MdwnB_kJmXAl-gJH0ribLAgDzqzUqW16EzrTPhoWJbAyLfrVkgVqER3A-kmF4dsPvCnSAtwtFXU3aUnqQ9ExxejNJwHzCLnEeJliZf-DkF_mNZ-n4rfnstTORZufe6X_D5-_DVE0jl4iZehoI360WJdZK1tFH7sesGKwzZJoi4zjYNjgdv6tGlngNtKhaWSTfPA0Kz2N51O14llq1SLp_QFEExbFYEkrDQugxYQ6GgywlD_hAAg2-f00s8MgASoJdXMtd2VzdC0yMJQI.m3u8 (404 Client Error: Not Found for url: https://video-weaver.fra02.hls.ttvnw.net/v1/playlist/CtIEZDm7NWyT-thnAUuZBiU69sb15zPPD9XZbKlLNGM8kvad-SgolHdrrncU1szlHTkQv-jjeNG8kWbTjajFkzJqI0vrnDaLh7BleaEqPGenNfXUYngYyPITTncELfAq8sjn0c3yFFFIao7CnoLfbDYC_NqOrDGJ0PAQGQaMnN3xrrIXEFxPOIYaIhtNMsbMZj7q40kVRD8lo4bYdB6IFxD5PQT2WPIJa2ugseh4Rn5NQw-Tf_pRQvhGVxinA_llE02hm-_pF0f2i-2KrXgvOFIqPqYC_7Nxc0OqDTYZsYW-PwFridPkWrT9R1Q7oWzepMFWBoXkhnlHFQGxW1-Efn3HGY5goejUggEFikuw97m4MU5SilCehYv8UbB8sDKsfUFTdwS1fz7Gp4BdcmgjcSj22Xn3oBC4V-JUVpJoV53gc_vTVrjlbHbGnBuF2MBbZz8Tazk53YmWgVU_QE2ZubX1qLCdlbwbRfckm23XADHpuC3sZyxS1CVh76m4ZXA4WGeW2y-LmriJnLj31h1awSpRADjJS0uDFOT8NPPiafZgXaTV6Y9-MdwnB_kJmXAl-gJH0ribLAgDzqzUqW16EzrTPhoWJbAyLfrVkgVqER3A-kmF4dsPvCnSAtwtFXU3aUnqQ9ExxejNJwHzCLnEeJliZf-DkF_mNZ-n4rfnstTORZufe6X_D5-_DVE0jl4iZehoI360WJdZK1tFH7sesGKwzZJoi4zjYNjgdv6tGlngNtKhaWSTfPA0Kz2N51O14llq1SLp_QFEExbFYEkrDQugxYQ6GgywlD_hAAg2-f00s8MgASoJdXMtd2VzdC0yMJQI.m3u8)
[stream.segmented][debug] Closing worker thread
[stream.segmented][debug] Closing writer thread
[cli][error] Try 1/1: Could not open stream <TwitchHLSStream ['hls', 'https://video-weaver.fra02.hls.ttvnw.net/v1/playlist/CtIEZDm7NWyT-thnAUuZBiU69sb15zPPD9XZbKlLNGM8kvad-SgolHdrrncU1szlHTkQv-jjeNG8kWbTjajFkzJqI0vrnDaLh7BleaEqPGenNfXUYngYyPITTncELfAq8sjn0c3yFFFIao7CnoLfbDYC_NqOrDGJ0PAQGQaMnN3xrrIXEFxPOIYaIhtNMsbMZj7q40kVRD8lo4bYdB6IFxD5PQT2WPIJa2ugseh4Rn5NQw-Tf_pRQvhGVxinA_llE02hm-_pF0f2i-2KrXgvOFIqPqYC_7Nxc0OqDTYZsYW-PwFridPkWrT9R1Q7oWzepMFWBoXkhnlHFQGxW1-Efn3HGY5goejUggEFikuw97m4MU5SilCehYv8UbB8sDKsfUFTdwS1fz7Gp4BdcmgjcSj22Xn3oBC4V-JUVpJoV53gc_vTVrjlbHbGnBuF2MBbZz8Tazk53YmWgVU_QE2ZubX1qLCdlbwbRfckm23XADHpuC3sZyxS1CVh76m4ZXA4WGeW2y-LmriJnLj31h1awSpRADjJS0uDFOT8NPPiafZgXaTV6Y9-MdwnB_kJmXAl-gJH0ribLAgDzqzUqW16EzrTPhoWJbAyLfrVkgVqER3A-kmF4dsPvCnSAtwtFXU3aUnqQ9ExxejNJwHzCLnEeJliZf-DkF_mNZ-n4rfnstTORZufe6X_D5-_DVE0jl4iZehoI360WJdZK1tFH7sesGKwzZJoi4zjYNjgdv6tGlngNtKhaWSTfPA0Kz2N51O14llq1SLp_QFEExbFYEkrDQugxYQ6GgywlD_hAAg2-f00s8MgASoJdXMtd2VzdC0yMJQI.m3u8', 'https://usher.ttvnw.net/api/channel/hls/paymoneywubby.m3u8?player=twitchweb&p=807153&type=any&allow_source=true&allow_audio_only=true&allow_spectre=false&sig=03af56e0a697256be328c92978b7f3d4993795b6&token=%7B%22adblock%22%3Afalse%2C%22authorization%22%3A%7B%22forbidden%22%3Afalse%2C%22reason%22%3A%22%22%7D%2C%22blackout_enabled%22%3Afalse%2C%22channel%22%3A%22paymoneywubby%22%2C%22channel_id%22%3A38251312%2C%22chansub%22%3A%7B%22restricted_bitrates%22%3A%5B%5D%2C%22view_until%22%3A1924905600%7D%2C%22ci_gb%22%3Afalse%2C%22geoblock_reason%22%3A%22%22%2C%22device_id%22%3Anull%2C%22expires%22%3A1701760815%2C%22extended_history_allowed%22%3Afalse%2C%22game%22%3A%22%22%2C%22hide_ads%22%3Afalse%2C%22https_required%22%3Atrue%2C%22mature%22%3Afalse%2C%22partner%22%3Afalse%2C%22platform%22%3A%22web%22%2C%22player_type%22%3A%22embed%22%2C%22private%22%3A%7B%22allowed_to_view%22%3Atrue%7D%2C%22privileged%22%3Afalse%2C%22role%22%3A%22%22%2C%22server_ads%22%3Atrue%2C%22show_ads%22%3Atrue%2C%22subscriber%22%3Afalse%2C%22turbo%22%3Afalse%2C%22user_id%22%3Anull%2C%22user_ip%22%3A%2285.67.13.26%22%2C%22version%22%3A2%7D&fast_bread=True']> (No data returned from stream)
error: Could not open stream <TwitchHLSStream ['hls', 'https://video-weaver.fra02.hls.ttvnw.net/v1/playlist/CtIEZDm7NWyT-thnAUuZBiU69sb15zPPD9XZbKlLNGM8kvad-SgolHdrrncU1szlHTkQv-jjeNG8kWbTjajFkzJqI0vrnDaLh7BleaEqPGenNfXUYngYyPITTncELfAq8sjn0c3yFFFIao7CnoLfbDYC_NqOrDGJ0PAQGQaMnN3xrrIXEFxPOIYaIhtNMsbMZj7q40kVRD8lo4bYdB6IFxD5PQT2WPIJa2ugseh4Rn5NQw-Tf_pRQvhGVxinA_llE02hm-_pF0f2i-2KrXgvOFIqPqYC_7Nxc0OqDTYZsYW-PwFridPkWrT9R1Q7oWzepMFWBoXkhnlHFQGxW1-Efn3HGY5goejUggEFikuw97m4MU5SilCehYv8UbB8sDKsfUFTdwS1fz7Gp4BdcmgjcSj22Xn3oBC4V-JUVpJoV53gc_vTVrjlbHbGnBuF2MBbZz8Tazk53YmWgVU_QE2ZubX1qLCdlbwbRfckm23XADHpuC3sZyxS1CVh76m4ZXA4WGeW2y-LmriJnLj31h1awSpRADjJS0uDFOT8NPPiafZgXaTV6Y9-MdwnB_kJmXAl-gJH0ribLAgDzqzUqW16EzrTPhoWJbAyLfrVkgVqER3A-kmF4dsPvCnSAtwtFXU3aUnqQ9ExxejNJwHzCLnEeJliZf-DkF_mNZ-n4rfnstTORZufe6X_D5-_DVE0jl4iZehoI360WJdZK1tFH7sesGKwzZJoi4zjYNjgdv6tGlngNtKhaWSTfPA0Kz2N51O14llq1SLp_QFEExbFYEkrDQugxYQ6GgywlD_hAAg2-f00s8MgASoJdXMtd2VzdC0yMJQI.m3u8', 'https://usher.ttvnw.net/api/channel/hls/paymoneywubby.m3u8?player=twitchweb&p=807153&type=any&allow_source=true&allow_audio_only=true&allow_spectre=false&sig=03af56e0a697256be328c92978b7f3d4993795b6&token=%7B%22adblock%22%3Afalse%2C%22authorization%22%3A%7B%22forbidden%22%3Afalse%2C%22reason%22%3A%22%22%7D%2C%22blackout_enabled%22%3Afalse%2C%22channel%22%3A%22paymoneywubby%22%2C%22channel_id%22%3A38251312%2C%22chansub%22%3A%7B%22restricted_bitrates%22%3A%5B%5D%2C%22view_until%22%3A1924905600%7D%2C%22ci_gb%22%3Afalse%2C%22geoblock_reason%22%3A%22%22%2C%22device_id%22%3Anull%2C%22expires%22%3A1701760815%2C%22extended_history_allowed%22%3Afalse%2C%22game%22%3A%22%22%2C%22hide_ads%22%3Afalse%2C%22https_required%22%3Atrue%2C%22mature%22%3Afalse%2C%22partner%22%3Afalse%2C%22platform%22%3A%22web%22%2C%22player_type%22%3A%22embed%22%2C%22private%22%3A%7B%22allowed_to_view%22%3Atrue%7D%2C%22privileged%22%3Afalse%2C%22role%22%3A%22%22%2C%22server_ads%22%3Atrue%2C%22show_ads%22%3Atrue%2C%22subscriber%22%3Afalse%2C%22turbo%22%3Afalse%2C%22user_id%22%3Anull%2C%22user_ip%22%3A%2285.67.13.26%22%2C%22version%22%3A2%7D&fast_bread=True']>, tried 1 times, exiting
[cli][info] Closing currently open stream...
```
Looks like the online/offline detection via the streaming access token acquirement doesn't work on this channel for some reason. This could be cause they are hosting another channel.
We'll have to get the channel's online status using another GQL query in addition to the access token. Twitch's GQL API is their private API and is undocumented. Let me have a look at what their site is doing...
That should work: `UseLive` / `639d5f11bfb8bf3053b424d9ef650d04c4ebb7d94711d644afb08fe9a0fad5d9`
**online**
```sh
curl -sSL -X POST -H 'Client-Id: kimne78kx3ncx6brgo4mv6wki5h1ko' -d '{
"operationName": "UseLive",
"variables": {
"channelLogin": "shroud"
},
"extensions": {
"persistedQuery": {
"version": 1,
"sha256Hash": "639d5f11bfb8bf3053b424d9ef650d04c4ebb7d94711d644afb08fe9a0fad5d9"
}
}
}' https://gql.twitch.tv/gql | jq
```
```json
{
"data": {
"user": {
"id": "37402112",
"login": "shroud",
"stream": {
"id": "43175975723",
"createdAt": "2023-12-04T23:07:43Z",
"__typename": "Stream"
},
"__typename": "User"
}
},
"extensions": {
"durationMilliseconds": 39,
"operationName": "UseLive",
"requestID": "..."
}
}
```
**offline**
```sh
curl -sSL -X POST -H 'Client-Id: kimne78kx3ncx6brgo4mv6wki5h1ko' -d '{
"operationName": "UseLive",
"variables": {
"channelLogin": "paymoneywubby"
},
"extensions": {
"persistedQuery": {
"version": 1,
"sha256Hash": "639d5f11bfb8bf3053b424d9ef650d04c4ebb7d94711d644afb08fe9a0fad5d9"
}
}
}' https://gql.twitch.tv/gql | jq
```
```json
{
"data": {
"user": {
"id": "38251312",
"login": "paymoneywubby",
"stream": null,
"__typename": "User"
}
},
"extensions": {
"durationMilliseconds": 39,
"operationName": "UseLive",
"requestID": "..."
}
}
```
Are reruns still a feature on Twitch? The plugin still implements a check if the channel's status is not `live`, but I don't know if it's actually useful anymore. Didn't they remove the rerun feature?
Not sure - in my case, the streams I record don't do reruns, so I wouldn't know, sorry.
See #5704 and give it a test:
https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#pull-request-feedback
Happy to confirm it works! Thank you!
```
[cli][debug] OS: Linux-5.10.0-26-amd64-x86_64-with-glibc2.31
[cli][debug] Python: 3.9.2
[cli][debug] OpenSSL: OpenSSL 1.1.1w 11 Sep 2023
[cli][debug] Streamlink: 6.4.2+3.gde794086
[cli][debug] Dependencies:
[cli][debug] certifi: 2020.6.20
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.3
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.19.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.23.1
[cli][debug] trio-websocket: 0.11.1
[cli][debug] typing-extensions: 4.8.0
[cli][debug] urllib3: 1.26.5
[cli][debug] websocket-client: 1.7.0
[cli][debug] Arguments:
[cli][debug] url=twitch.tv/paymoneywubby
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --output=/disk1/share1/twitch/{time}.ts
[cli][debug] --retry-streams=5.0
[cli][info] Found matching plugin twitch for URL twitch.tv/paymoneywubby
[plugins.twitch][error] Channel is offline
[cli][info] Waiting for streams, retrying every 5.0 second(s)
[plugins.twitch][error] Channel is offline
[plugins.twitch][error] Channel is offline
[plugins.twitch][error] Channel is offline
Interrupted! Exiting...
```
Let's keep this open until #5704 has been merged.
Whenever i try to load a stream it just comes up with this:
https://i.imgur.com/X1WNJWB.png
Seems to be working now.
I don't see any issues with the plugin. Twitch live streams are working fine, including ad filtering and low latency streaming, as well as VODs. I've checked various channels and qualities.
What you have posted is not a debug log. You completely ignored the issue form/template, so this issue doesn't have any useful information at all apart from the error message Twitch is spitting out. I don't even know which channel you're attempting to watch.
As you can see from the error message, Twitch doesn't let you access the HLS playlist because this specific quality hasn't been encoded (yet?).
Streamlink `6.3.0` fixed the error handling of the Twitch plugin when resolving HLS playlists, which you can read in the changelog:
https://streamlink.github.io/changelog.html#streamlink-6-3-0-2023-10-25
This was fixed because of geo-restricted streams on Twitch with Amazon Prime-Video stuff, exclusive to the US area.
> started flooding
Nothing is flooding unless you've set `--retry-streams`. Streamlink's CLI will keep resolving the streams on error when set.
https://streamlink.github.io/cli.html#cmdoption-retry-streams
----
> Whenever i try to load a stream it just comes up with this:
Please stop posting Twitch GUI stuff here on this issue tracker. It doesn't belong here. This image also doesn't say anything, as well as your post. My guess however is that your player closes on its own immediately when it receives its input. Otherwise you'd see a Streamlink error message. This is a regular exit of the playback, at least from the perspective from Streamlink. The Twitch GUI is just a launcher and isn't involved at all here.
> > started flooding
>
> Nothing is flooding unless you've set `--retry-streams`. Streamlink's CLI will keep resolving the streams on error when set. https://streamlink.github.io/cli.html#cmdoption-retry-streams
>
yeah and that is new behavior. since its setup to poll the channel for streams to record there will be a lot of those messages. in the past it would retry silently | 2023-12-05T08:43:43 |
streamlink/streamlink | 5,708 | streamlink__streamlink-5708 | [
"5707"
] | e58fe2433c9ac6bff8f7ba0d2f8add2cc242e108 | diff --git a/src/streamlink/plugins/twitch.py b/src/streamlink/plugins/twitch.py
--- a/src/streamlink/plugins/twitch.py
+++ b/src/streamlink/plugins/twitch.py
@@ -487,26 +487,6 @@ def clips(self, clipname):
),
))
- def stream_metadata(self, channel):
- query = self._gql_persisted_query(
- "StreamMetadata",
- "1c719a40e481453e5c48d9bb585d971b8b372f8ebb105b17076722264dfa5b3e",
- channelLogin=channel,
- )
-
- return self.call(query, schema=validate.Schema(
- {
- "data": {
- "user": validate.none_or_all({
- "stream": validate.none_or_all({
- "id": str,
- }),
- }),
- },
- },
- validate.get(("data", "user")),
- ))
-
class TwitchClientIntegrity:
URL_P_SCRIPT = "https://k.twitchcdn.net/149e9513-01fa-4fb0-aad4-566afd725d1b/2d206a39-8ed7-437e-a3be-862e0f06eea3/p.js"
@@ -816,21 +796,7 @@ def _access_token(self, is_live, channel_or_vod):
return sig, token, restricted_bitrates
- def _check_is_live(self) -> bool:
- data = self.api.stream_metadata(self.channel)
- if data is None:
- log.error("Unknown channel")
- return False
- if data["stream"] is None:
- log.error("Channel is offline")
- return False
-
- return True
-
def _get_hls_streams_live(self):
- if not self._check_is_live():
- return
-
# only get the token once the channel has been resolved
log.debug(f"Getting live HLS streams for {self.channel}")
self.session.http.headers.update({
@@ -863,6 +829,7 @@ def _get_hls_streams(self, url, restricted_bitrates, **extra_params):
self.session,
url,
start_offset=time_offset,
+ check_streams=True,
disable_ads=self.get_option("disable-ads"),
low_latency=self.get_option("low-latency"),
**extra_params,
@@ -871,7 +838,6 @@ def _get_hls_streams(self, url, restricted_bitrates, **extra_params):
# TODO: fix the "err" attribute set by HTTPSession.request()
orig = getattr(err, "err", None)
if isinstance(orig, HTTPError) and orig.response.status_code >= 400:
- error = None
with suppress(PluginError):
error = validate.Schema(
validate.parse_json(),
@@ -881,7 +847,7 @@ def _get_hls_streams(self, url, restricted_bitrates, **extra_params):
}],
validate.get((0, "error")),
).validate(orig.response.text)
- log.error(error or "Could not access HLS playlist")
+ log.error(error or "Could not access HLS playlist")
return
raise PluginError(err) from err
| diff --git a/tests/plugins/test_twitch.py b/tests/plugins/test_twitch.py
--- a/tests/plugins/test_twitch.py
+++ b/tests/plugins/test_twitch.py
@@ -396,53 +396,6 @@ def test_hls_low_latency_no_ads_reload_time(self):
assert self.thread.reader.worker.playlist_reload_time == pytest.approx(23 / 3)
-class TestTwitchIsLive:
- @pytest.fixture()
- def plugin(self, request: pytest.FixtureRequest):
- return Twitch(Streamlink(), "https://twitch.tv/channelname")
-
- @pytest.fixture()
- def mock(self, request: pytest.FixtureRequest, requests_mock: rm.Mocker):
- mock = requests_mock.post("https://gql.twitch.tv/gql", **getattr(request, "param", {"json": {}}))
- yield mock
- assert mock.call_count > 0
- payload = mock.last_request.json() # type: ignore[union-attr]
- assert tuple(sorted(payload.keys())) == ("extensions", "operationName", "variables")
- assert payload.get("operationName") == "StreamMetadata"
- assert payload.get("extensions") == {
- "persistedQuery": {
- "sha256Hash": "1c719a40e481453e5c48d9bb585d971b8b372f8ebb105b17076722264dfa5b3e",
- "version": 1,
- },
- }
- assert payload.get("variables") == {"channelLogin": "channelname"}
-
- @pytest.mark.parametrize(("mock", "expected", "log"), [
- pytest.param(
- {"json": {"data": {"user": None}}},
- False,
- [("streamlink.plugins.twitch", "error", "Unknown channel")],
- id="no-user",
- ),
- pytest.param(
- {"json": {"data": {"user": {"stream": None}}}},
- False,
- [("streamlink.plugins.twitch", "error", "Channel is offline")],
- id="no-stream",
- ),
- pytest.param(
- {"json": {"data": {"user": {"stream": {"id": "1234567890"}}}}},
- True,
- [],
- id="is-live",
- ),
- ], indirect=["mock"])
- def test_is_live(self, plugin: Twitch, caplog: pytest.LogCaptureFixture, mock: rm.Mocker, expected: bool, log: list):
- caplog.set_level(1, "streamlink")
- assert plugin._check_is_live() is expected
- assert [(record.name, record.levelname, record.message) for record in caplog.records] == log
-
-
class TestTwitchAPIAccessToken:
@pytest.fixture(autouse=True)
def _client_integrity_token(self, monkeypatch: pytest.MonkeyPatch):
@@ -676,7 +629,7 @@ def plugin(self, request: pytest.FixtureRequest, requests_mock: rm.Mocker, sessi
},
nullcontext(),
None,
- [("streamlink.plugins.twitch", "error", "Could not access HLS playlist")],
+ [],
id="non-json error response",
),
], indirect=["plugin"])
| plugins.twitch: Returns "Channel is offline" for short period after a stream has started
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
6.4.2+6.ge58fe243
### Description
After https://github.com/streamlink/streamlink/pull/5704, streams will now return "Channel is offline" for a short period after the stream has started, even when the stream is viewable in a web browser. The period seems to vary but I've seen it as high as around 30 seconds.
You should be able to reproduce this using a personal Twitch channel, open the channel in a browser window, prepare a sample streamlink command (`streamlink -o /dev/null https://twitch.tv/channel best`), then start streaming to the channel. The stream should start autoplaying in the browser a short time later, once this happens attempt to run the streamlink command. It should fail with "Channel is offline", it will keep returning this for another 15~30 seconds in my experience before working properly.
Before the linked commit, streamlink would be able to play a stream immediately after it started, the same time it was viewable in the browser.
### Debug log
```text
[13:22:47.708671][cli][debug] OS: Linux-6.1.65-1-lts-x86_64-with-glibc2.38
[13:22:47.708918][cli][debug] Python: 3.11.6
[13:22:47.709048][cli][debug] OpenSSL: OpenSSL 3.1.4 24 Oct 2023
[13:22:47.709126][cli][debug] Streamlink: 6.4.2+6.ge58fe243
[13:22:47.709739][cli][debug] Dependencies:
[13:22:47.711347][cli][debug] certifi: 2023.11.17
[13:22:47.711896][cli][debug] isodate: 0.6.1
[13:22:47.712349][cli][debug] lxml: 4.9.3
[13:22:47.713059][cli][debug] pycountry: 22.3.5
[13:22:47.713634][cli][debug] pycryptodome: 3.19.0
[13:22:47.714240][cli][debug] PySocks: 1.7.1
[13:22:47.714741][cli][debug] requests: 2.31.0
[13:22:47.715192][cli][debug] trio: 0.23.1
[13:22:47.715626][cli][debug] trio-websocket: 0.11.1
[13:22:47.716044][cli][debug] typing-extensions: 4.8.0
[13:22:47.716471][cli][debug] urllib3: 2.1.0
[13:22:47.717358][cli][debug] websocket-client: 1.7.0
[13:22:47.717598][cli][debug] Arguments:
[13:22:47.717679][cli][debug] url=https://www.twitch.tv/<channel>
[13:22:47.717743][cli][debug] stream=['best']
[13:22:47.717811][cli][debug] --loglevel=all
[13:22:47.717869][cli][debug] --logfile=2023_12_05_21_22_47_(UTC).log
[13:22:47.717935][cli][debug] --output=2023_12_05_21_22_47_(UTC).ts
[13:22:47.718014][cli][debug] --force-progress=True
[13:22:47.718085][cli][debug] --retry-streams=5.0
[13:22:47.718142][cli][debug] --retry-max=12
[13:22:47.718195][cli][debug] --retry-open=10
[13:22:47.718252][cli][debug] --stream-segment-attempts=20
[13:22:47.718305][cli][debug] --stream-segment-threads=10
[13:22:47.718359][cli][debug] --stream-segment-timeout=60.0
[13:22:47.718413][cli][debug] --stream-timeout=240.0
[13:22:47.718469][cli][debug] --hls-playlist-reload-attempts=10
[13:22:47.718554][cli][debug] --hls-playlist-reload-time=segment
[13:22:47.718615][cli][debug] --hls-live-restart=True
[13:22:47.718684][cli][debug] --webbrowser=False
[13:22:47.718755][cli][debug] --twitch-disable-hosting=True
[13:22:47.719307][cli][info] Found matching plugin twitch for URL https://www.twitch.tv/<channel>
[13:22:47.860846][plugins.twitch][error] Channel is offline
[13:22:47.861003][cli][info] Waiting for streams, retrying every 5.0 second(s)
[13:22:52.934465][plugins.twitch][error] Channel is offline
[13:22:58.009304][plugins.twitch][debug] Getting live HLS streams for <channel>
[13:22:58.106980][plugins.twitch][debug] {'adblock': False, 'geoblock_reason': '', 'hide_ads': False, 'server_ads': True, 'show_ads': True}
[13:22:58.153457][utils.l10n][debug] Language code: en_US
[13:22:58.306960][cli][info] Available streams: audio_only, 720p60 (worst, best)
[13:22:58.307176][cli][info] Opening stream: 720p60 (hls)
```
| This is because their GQL API has a slight delay, same issue with the stream metadata retrieval when polling the stream previously using `--retry-streams` (#4411 and all the linked duplicate threads).
How would you suggest fixing this otherwise? The reason why the API gets checked for the live status now is that just getting the access token results in streams being returned incorrectly for offline streams, hence the change. Then there's the issue with geo-blocked content on the `primevideo` channel, which was the reason why the error handling got changed recently. Simply checking for a 404 HTTP status code when trying to access the multivariant or media playlists doesn't help. The only other idea I have is checking if other API endpoints don't have this delay.
I don't think this slight delay is an issue. It even fixes said metadata retrieval issue.
I think this issue would cause a lot more inconvenience than the extremely rare issue it was meant to fix, I've been using streamlink on Twitch channels for years and this is the first time I've ever seen a channel with a weird broken playlist like that. Even the Twitch site doesn't handle it correctly, it just infinitely loads the video and never says the channel is offline.
It seems like the individual variant playlists properly returns a `404` code, even when the multivariant returns a `200`. Maybe a `404` on the first variant playlist fetch could signal an offline stream? I don't know if this would lead to any false positives though, would need testing probably.
If the is-live API check gets removed, then the following things need to be checked:
- **online channels**
Nothing interesting here...
- **invalid/unknown channels**
This is covered by the access token API response, so HLS stream error handling is not involved here.
- **`4xx` multivariant responses**
`4xx` HTTP responses when accessing the multivariant playlist **sometimes** include JSON data with error messages.
Previously, this JSON data has been ignored, but I've updated the error handling recently in #5591 because of geo-blocked streams like `primevideo`, so a better error message could be logged.
However, this also negatively affected regular offline streams in some cases, and it has already caused confusion (#5656).
- **regular offline channels**
Currently logs one of these garbage error messages:
- `twirp error not_found: transcode does not exist` (from the JSON data)
- `Could not access HLS playlist` (no JSON data)
- **geo-blocked streams**
Useful error logging:
- `Content Restricted In Region`
- **`200` multivariant playlist responses with `4xx` media playlist responses**
This is currently the case on the `paymoneywubby` channel, which was the motivation for the is-live API check. I don't think this is an edge case. This is probably because of some offline-hosting settings on this channel.
Setting `check_streams=True` when parsing the multivariant playlist allows detecting this case, but this comes at the cost of delaying all stream listings and individual stream starts, because each media playlist gets checked **consecutively**, meaning 6 or more HTTP requests will be made in order and each response gets awaited until we know that any channel is actually live or not. | 2023-12-05T23:31:56 |
streamlink/streamlink | 5,711 | streamlink__streamlink-5711 | [
"5710"
] | a169d5b89d1181fc28ebaca4594cfd709fcaf527 | diff --git a/src/streamlink/plugins/wasd.py b/src/streamlink/plugins/wasd.py
deleted file mode 100644
--- a/src/streamlink/plugins/wasd.py
+++ /dev/null
@@ -1,87 +0,0 @@
-"""
-$description Russian live-streaming social platform.
-$url wasd.tv
-$type live
-"""
-
-import logging
-import re
-
-from streamlink.plugin import Plugin, PluginError, pluginmatcher
-from streamlink.plugin.api import validate
-from streamlink.stream.hls import HLSStream
-
-
-log = logging.getLogger(__name__)
-
-
-@pluginmatcher(re.compile(
- r"https?://(?:www\.)?wasd\.tv/(?P<nickname>[^/]+)/?$",
-))
-class WASD(Plugin):
- _media_schema = validate.Schema({
- "user_id": int,
- "media_container_online_status": str,
- "media_container_status": str,
- "media_container_streams": [{
- "stream_media": [{
- "media_id": int,
- "media_meta": {
- "media_url": validate.any(str, None),
- "media_archive_url": validate.any(str, None),
- },
- "media_status": validate.any("STOPPED", "RUNNING"),
- "media_type": "HLS",
- }],
- }],
- })
- _api_schema = validate.Schema({
- "result":
- validate.any(
- _media_schema,
- validate.all(list,
- validate.get(0),
- _media_schema),
- [],
- ),
- }, validate.get("result"))
- _api_nicknames_schema = validate.Schema({
- "result": {
- "channel_id": int,
- },
- }, validate.get("result"), validate.get("channel_id"))
-
- def _get_streams(self):
- nickname = self.match.group("nickname")
- res = self.session.http.get(f"https://wasd.tv/api/channels/nicknames/{nickname}")
- channel_id = self.session.http.json(res, schema=self._api_nicknames_schema)
-
- res = self.session.http.get(
- "https://wasd.tv/api/v2/media-containers",
- params={
- "media_container_status": "RUNNING",
- "limit": "1",
- "offset": "0",
- "channel_id": channel_id,
- "media_container_type": "SINGLE,COOP",
- },
- )
-
- json_res = self.session.http.json(res, schema=self._api_schema)
- log.trace("{0!r}".format(json_res))
- if not json_res:
- raise PluginError("No data returned from URL={0}".format(res.url))
-
- for stream in json_res["media_container_streams"]:
- log.debug("media_container_status: {0}, media_container_online_status: {1}".format(
- json_res["media_container_status"], json_res["media_container_online_status"]))
- for stream_media in stream["stream_media"]:
- if stream_media["media_status"] == "STOPPED":
- hls_url = stream_media["media_meta"]["media_archive_url"]
- else:
- hls_url = stream_media["media_meta"]["media_url"]
-
- yield from HLSStream.parse_variant_playlist(self.session, hls_url).items()
-
-
-__plugin__ = WASD
| diff --git a/tests/plugins/test_wasd.py b/tests/plugins/test_wasd.py
deleted file mode 100644
--- a/tests/plugins/test_wasd.py
+++ /dev/null
@@ -1,17 +0,0 @@
-from streamlink.plugins.wasd import WASD
-from tests.plugins import PluginCanHandleUrl
-
-
-class TestPluginCanHandleUrlWasd(PluginCanHandleUrl):
- __plugin__ = WASD
-
- should_match = [
- "https://wasd.tv/channel",
- "https://wasd.tv/channel/",
- ]
-
- should_not_match = [
- "https://wasd.tv/channel/12345",
- "https://wasd.tv/channel/12345/videos/67890",
- "https://wasd.tv/voodik/videos?record=123456",
- ]
| plugins.wasd: service gone
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
6.4.2
### Description
A few days ago, the service [gone](https://mts.ru/personal/novosti/2023-12-05/vstrechajte-polzovatelskuyu-videoplatformu-nuum). Now this [nuum.ru](https://nuum.ru).
Though we could easily replace the plugin, but I'm not sure it's worth adding it to upstream, because it's a beta version.
<details>
```diff
diff --git a/src/streamlink/plugins/wasd.py b/src/streamlink/plugins/wasd.py
index 7d61304e..656a16eb 100644
--- a/src/streamlink/plugins/wasd.py
+++ b/src/streamlink/plugins/wasd.py
@@ -16,7 +16,7 @@ log = logging.getLogger(__name__)
@pluginmatcher(re.compile(
- r"https?://(?:www\.)?wasd\.tv/(?P<nickname>[^/]+)/?$",
+ r"https?://(?:www\.)?nuum\.ru/channel/(?P<nickname>[^/]+)/?$",
))
class WASD(Plugin):
_media_schema = validate.Schema({
@@ -53,11 +53,11 @@ class WASD(Plugin):
def _get_streams(self):
nickname = self.match.group("nickname")
- res = self.session.http.get(f"https://wasd.tv/api/channels/nicknames/{nickname}")
+ res = self.session.http.get(f"https://nuum.ru/api/channels/nicknames/{nickname}")
channel_id = self.session.http.json(res, schema=self._api_nicknames_schema)
res = self.session.http.get(
- "https://wasd.tv/api/v2/media-containers",
+ "https://nuum.ru/api/v2/media-containers",
params={
"media_container_status": "RUNNING",
"limit": "1",
```
</details>
### Debug log
| 2023-12-07T12:45:37 |
|
streamlink/streamlink | 5,725 | streamlink__streamlink-5725 | [
"5724"
] | bebc2ae181ebccaf6a1b569570bb625383a1e8e2 | diff --git a/src/streamlink/plugins/gulli.py b/src/streamlink/plugins/gulli.py
--- a/src/streamlink/plugins/gulli.py
+++ b/src/streamlink/plugins/gulli.py
@@ -11,79 +11,37 @@
from streamlink.plugin import Plugin, pluginmatcher
from streamlink.plugin.api import validate
from streamlink.stream.hls import HLSStream
-from streamlink.stream.http import HTTPStream
log = logging.getLogger(__name__)
-@pluginmatcher(re.compile(
- r"https?://replay\.gulli\.fr/(?:Direct|.+/(?P<video_id>VOD\d+))",
-))
+@pluginmatcher(
+ name="live",
+ pattern=re.compile(r"https?://replay\.gulli\.fr/Direct"),
+)
+@pluginmatcher(
+ name="vod",
+ pattern=re.compile(r"https?://replay\.gulli\.fr/.+-(?P<video_id>(?i:vod)\d+)$"),
+)
class Gulli(Plugin):
LIVE_PLAYER_URL = "https://replay.gulli.fr/jwplayer/embedstreamtv"
VOD_PLAYER_URL = "https://replay.gulli.fr/jwplayer/embed/{0}"
- _playlist_re = re.compile(r"sources: (\[.+?\])", re.DOTALL)
- _vod_video_index_re = re.compile(r"jwplayer\(idplayer\).playlistItem\((?P<video_index>[0-9]+)\)")
- _mp4_bitrate_re = re.compile(r".*_(?P<bitrate>[0-9]+)\.mp4")
-
- _video_schema = validate.Schema(
- validate.all(
- validate.transform(lambda x: re.sub(r'"?file"?:\s*[\'"](.+?)[\'"],?', r'"file": "\1"', x, flags=re.DOTALL)),
- validate.transform(lambda x: re.sub(r'"?\w+?"?:\s*function\b.*?(?<={).*(?=})', "", x, flags=re.DOTALL)),
- validate.parse_json(),
- [
- validate.Schema({
- "file": validate.url(),
- }),
- ],
- ),
- )
-
def _get_streams(self):
- video_id = self.match.group("video_id")
- if video_id is not None:
- # VOD
- live = False
- player_url = self.VOD_PLAYER_URL.format(video_id)
- else:
- # Live
- live = True
+ if self.matches["live"]:
player_url = self.LIVE_PLAYER_URL
+ else:
+ player_url = self.VOD_PLAYER_URL.format(self.match["video_id"])
- res = self.session.http.get(player_url)
- playlist = re.findall(self._playlist_re, res.text)
- index = 0
- if not live:
- # Get the index for the video on the playlist
- match = self._vod_video_index_re.search(res.text)
- if match is None:
- return
- index = int(match.group("video_index"))
-
- if not playlist:
+ video_url = self.session.http.get(player_url, schema=validate.Schema(
+ validate.parse_html(),
+ validate.xml_xpath_string(".//video-js[1]/source[@src][@type='application/x-mpegURL'][1]/@src"),
+ ))
+ if not video_url:
return
- videos = self._video_schema.validate(playlist[index])
-
- for video in videos:
- video_url = video["file"]
-
- # Ignore non-supported MSS streams
- if "isml/Manifest" in video_url:
- continue
- try:
- if ".m3u8" in video_url:
- yield from HLSStream.parse_variant_playlist(self.session, video_url).items()
- elif ".mp4" in video_url:
- match = self._mp4_bitrate_re.match(video_url)
- bitrate = "vod" if match is None else f"{match.group('bitrate')}k"
- yield bitrate, HTTPStream(self.session, video_url)
- except OSError as err:
- if "403 Client Error" in str(err):
- log.error("Failed to access stream, may be due to geo-restriction")
- raise
+ return HLSStream.parse_variant_playlist(self.session, video_url)
__plugin__ = Gulli
| diff --git a/tests/plugins/test_gulli.py b/tests/plugins/test_gulli.py
--- a/tests/plugins/test_gulli.py
+++ b/tests/plugins/test_gulli.py
@@ -6,10 +6,22 @@ class TestPluginCanHandleUrlGulli(PluginCanHandleUrl):
__plugin__ = Gulli
should_match = [
- "http://replay.gulli.fr/Direct",
- "http://replay.gulli.fr/dessins-animes/My-Little-Pony-les-amies-c-est-magique/VOD68328764799000",
- "https://replay.gulli.fr/emissions/In-Ze-Boite2/VOD68639028668000",
- "https://replay.gulli.fr/series/Power-Rangers-Dino-Super-Charge/VOD68612908435000",
+ (
+ "live",
+ "https://replay.gulli.fr/Direct",
+ ),
+ (
+ "vod",
+ "https://replay.gulli.fr/dessins-animes/Bob-l-eponge-s10/bob-l-eponge-s10-e05-un-bon-gros-dodo-vod69736581475000",
+ ),
+ (
+ "vod",
+ "https://replay.gulli.fr/emissions/Animaux-VIP-une-bete-de-reno-s02/animaux-vip-une-bete-de-reno-s02-e09-la-taniere-du-dragon-vod69634261609000",
+ ),
+ (
+ "vod",
+ "https://replay.gulli.fr/series/Black-Panther-Dangers-au-Wakanda/black-panther-dangers-au-wakanda-black-panther-dangers-au-wakanda-vod69941412154000",
+ ),
]
should_not_match = [
| plugins.gulli: No playable streams found on this URL: https://replay.gulli.fr/Direct
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
streamlink 6.4.2
### Description
The gulli plugin can't find the stream on the gulli page: https://replay.gulli.fr/Direct
Opening the page from a browser will auto-play the video.
### Debug log
```text
/ # streamlink --loglevel debug https://replay.gulli.fr/Direct
[cli][info] streamlink is running as root! Be careful!
[cli][debug] OS: Linux-6.1.21-v8+-aarch64-with
[cli][debug] Python: 3.9.13
[cli][debug] OpenSSL: OpenSSL 1.1.1o 3 May 2022
[cli][debug] Streamlink: 6.4.2
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.11.17
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.3
[cli][debug] pycountry: 23.12.11
[cli][debug] pycryptodome: 3.19.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.23.2
[cli][debug] trio-websocket: 0.11.1
[cli][debug] typing-extensions: 4.9.0
[cli][debug] urllib3: 2.1.0
[cli][debug] websocket-client: 1.7.0
[cli][debug] Arguments:
[cli][debug] url=https://replay.gulli.fr/Direct
[cli][debug] --loglevel=debug
[cli][info] Found matching plugin gulli for URL https://replay.gulli.fr/Direct
error: No playable streams found on this URL: https://replay.gulli.fr/Direct
```
| 2023-12-15T15:33:06 |
|
streamlink/streamlink | 5,731 | streamlink__streamlink-5731 | [
"5730"
] | 60fca3485a1a4a2d43dca43e3cfb14dd7cb6ba4a | diff --git a/src/streamlink/plugins/chzzk.py b/src/streamlink/plugins/chzzk.py
new file mode 100644
--- /dev/null
+++ b/src/streamlink/plugins/chzzk.py
@@ -0,0 +1,196 @@
+"""
+$description South Korean live-streaming platform for gaming, entertainment, and other creative content. Owned by Naver.
+$url chzzk.naver.com
+$type live, vod
+"""
+
+import logging
+import re
+
+from streamlink.plugin import Plugin, pluginmatcher
+from streamlink.plugin.api import validate
+from streamlink.stream.dash import DASHStream
+from streamlink.stream.hls import HLSStream
+
+
+log = logging.getLogger(__name__)
+
+
+class ChzzkAPI:
+ _CHANNELS_LIVE_DETAIL_URL = "https://api.chzzk.naver.com/service/v2/channels/{channel_id}/live-detail"
+ _VIDEOS_URL = "https://api.chzzk.naver.com/service/v2/videos/{video_id}"
+
+ def __init__(self, session):
+ self._session = session
+
+ def _query_api(self, url, *schemas):
+ return self._session.http.get(
+ url,
+ acceptable_status=(200, 404),
+ schema=validate.Schema(
+ validate.parse_json(),
+ validate.any(
+ validate.all(
+ {
+ "code": int,
+ "message": str,
+ },
+ validate.transform(lambda data: ("error", data["message"])),
+ ),
+ validate.all(
+ {
+ "code": 200,
+ "content": dict,
+ },
+ validate.get("content"),
+ *schemas,
+ validate.transform(lambda data: ("success", data)),
+ ),
+ ),
+ ),
+ )
+
+ def get_live_detail(self, channel_id):
+ return self._query_api(
+ self._CHANNELS_LIVE_DETAIL_URL.format(channel_id=channel_id),
+ {
+ "status": str,
+ "liveId": int,
+ "liveTitle": validate.any(str, None),
+ "liveCategory": validate.any(str, None),
+ "adult": bool,
+ "channel": validate.all(
+ {"channelName": str},
+ validate.get("channelName"),
+ ),
+ "livePlaybackJson": validate.none_or_all(
+ str,
+ validate.parse_json(),
+ {
+ "media": [
+ validate.all(
+ {
+ "mediaId": str,
+ "protocol": str,
+ "path": validate.url(),
+ },
+ validate.union_get(
+ "mediaId",
+ "protocol",
+ "path",
+ ),
+ ),
+ ],
+ },
+ validate.get("media"),
+ ),
+ },
+ validate.union_get(
+ "livePlaybackJson",
+ "status",
+ "liveId",
+ "channel",
+ "liveCategory",
+ "liveTitle",
+ "adult",
+ ),
+ )
+
+ def get_videos(self, video_id):
+ return self._query_api(
+ self._VIDEOS_URL.format(video_id=video_id),
+ {
+ "inKey": validate.any(str, None),
+ "videoId": validate.any(str, None),
+ "videoTitle": validate.any(str, None),
+ "videoCategory": validate.any(str, None),
+ "adult": bool,
+ "channel": validate.all(
+ {"channelName": str},
+ validate.get("channelName"),
+ ),
+ },
+ validate.union_get(
+ "inKey",
+ "videoId",
+ "channel",
+ "videoCategory",
+ "videoTitle",
+ "adult",
+ ),
+ )
+
+
+@pluginmatcher(
+ name="live",
+ pattern=re.compile(
+ r"https?://chzzk\.naver\.com/live/(?P<channel_id>[^/?]+)",
+ ),
+)
+@pluginmatcher(
+ name="video",
+ pattern=re.compile(
+ r"https?://chzzk\.naver\.com/video/(?P<video_id>[^/?]+)",
+ ),
+)
+class Chzzk(Plugin):
+ _API_VOD_PLAYBACK_URL = "https://apis.naver.com/neonplayer/vodplay/v2/playback/{video_id}?key={in_key}"
+
+ _STATUS_OPEN = "OPEN"
+
+ def __init__(self, *args, **kwargs):
+ super().__init__(*args, **kwargs)
+ self._api = ChzzkAPI(self.session)
+
+ def _get_live(self, channel_id):
+ datatype, data = self._api.get_live_detail(channel_id)
+ if datatype == "error":
+ log.error(data)
+ return
+
+ media, status, self.id, self.author, self.category, self.title, adult = data
+ if status != self._STATUS_OPEN:
+ log.error("The stream is unavailable")
+ return
+ if media is None:
+ log.error(f"This stream is for {'adults only' if adult else 'unavailable'}")
+ return
+
+ for media_id, media_protocol, media_path in media:
+ if media_protocol == "HLS" and media_id == "HLS":
+ return HLSStream.parse_variant_playlist(
+ self.session,
+ media_path,
+ channel_id=channel_id,
+ ffmpeg_options={"copyts": True},
+ )
+
+ def _get_video(self, video_id):
+ datatype, data = self._api.get_videos(video_id)
+ if datatype == "error":
+ log.error(data)
+ return
+
+ self.id = video_id
+ in_key, vod_id, self.author, self.category, self.title, adult = data
+
+ if in_key is None or vod_id is None:
+ log.error(f"This stream is for {'adults only' if adult else 'unavailable'}")
+ return
+
+ for name, stream in DASHStream.parse_manifest(
+ self.session,
+ self._API_VOD_PLAYBACK_URL.format(video_id=vod_id, in_key=in_key),
+ headers={"Accept": "application/dash+xml"},
+ ).items():
+ if stream.video_representation.mimeType == "video/mp2t":
+ yield name, stream
+
+ def _get_streams(self):
+ if self.matches["live"]:
+ return self._get_live(self.match["channel_id"])
+ elif self.matches["video"]:
+ return self._get_video(self.match["video_id"])
+
+
+__plugin__ = Chzzk
| diff --git a/tests/plugins/test_chzzk.py b/tests/plugins/test_chzzk.py
new file mode 100644
--- /dev/null
+++ b/tests/plugins/test_chzzk.py
@@ -0,0 +1,11 @@
+from streamlink.plugins.chzzk import Chzzk
+from tests.plugins import PluginCanHandleUrl
+
+
+class TestPluginCanHandleUrlChzzk(PluginCanHandleUrl):
+ __plugin__ = Chzzk
+
+ should_match_groups = [
+ (("live", "https://chzzk.naver.com/live/CHANNEL_ID"), {"channel_id": "CHANNEL_ID"}),
+ (("video", "https://chzzk.naver.com/video/VIDEO_ID"), {"video_id": "VIDEO_ID"}),
+ ]
| CHZZK, Naverβs new Korean streaming plateform
### Checklist
- [X] This is a plugin request and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin requests](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+request%22)
### Description
A new streaming plateform from the Korean company Naver called "[CHZZK](https://chzzk.naver.com/)" is likely to quickly receive traction from Korean streamers that canβt stay on Twitch.
Itβs still in beta though, so it might change a little, but it would be nice to explore the possibility to have a plugin started for this streaming plateform.
### Input URLs
https://chzzk.naver.com/live/17f0cfcba4ff608de5eabb5110d134d0
https://chzzk.naver.com/live/c68b8ef525fb3d2fa146344d84991753
https://chzzk.naver.com/live/4b2f27574a2e60d15bd53f94cbfc4066
| 2023-12-19T15:07:57 |
|
streamlink/streamlink | 5,739 | streamlink__streamlink-5739 | [
"5738"
] | 1bb5239c3b8f7feb34a927b4846004ce070a4783 | diff --git a/src/streamlink/plugins/zattoo.py b/src/streamlink/plugins/zattoo.py
--- a/src/streamlink/plugins/zattoo.py
+++ b/src/streamlink/plugins/zattoo.py
@@ -257,7 +257,11 @@ def _watch(self):
log.debug(f"Found data for {stream_type}")
if stream_type == "hls7":
for url in data["stream"]["watch_urls"]:
- yield from HLSStream.parse_variant_playlist(self.session, url["url"]).items()
+ yield from HLSStream.parse_variant_playlist(
+ self.session,
+ url["url"],
+ ffmpeg_options={"copyts": True},
+ ).items()
elif stream_type == "dash":
for url in data["stream"]["watch_urls"]:
yield from DASHStream.parse_manifest(self.session, url["url"]).items()
| plugins.zattoo: Audio and video sometimes out of sync in HLS7 streams
### Checklist
- [X] This is a bug report and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed bug reports](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22bug%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
streamlink 6.5.0+2.g1bb5239c
### Description
About every 20th time when starting watching, audio and video are out of sync. Audio is then ahead about 5 seconds (maybe one segment length?). This happens for me when using the zattoo plugin with hls7 streams, but I suppose that the problem is more related to the hls stream handling and not to the zattoo plugin.
### Debug log
```text
[13:13:40.444581][cli][debug] OS: Linux-6.5.0-0.deb12.4-amd64-x86_64-with-glibc2.36
[13:13:40.444767][cli][debug] Python: 3.11.2
[13:13:40.444854][cli][debug] OpenSSL: OpenSSL 3.0.11 19 Sep 2023
[13:13:40.444925][cli][debug] Streamlink: 6.5.0+2.g1bb5239c
[13:13:40.445535][cli][debug] Dependencies:
[13:13:40.446407][cli][debug] certifi: 2023.11.17
[13:13:40.446886][cli][debug] isodate: 0.6.1
[13:13:40.447291][cli][debug] lxml: 4.9.4
[13:13:40.447874][cli][debug] pycountry: 23.12.11
[13:13:40.448261][cli][debug] pycryptodome: 3.19.0
[13:13:40.448793][cli][debug] PySocks: 1.7.1
[13:13:40.449207][cli][debug] requests: 2.31.0
[13:13:40.449600][cli][debug] trio: 0.23.2
[13:13:40.449978][cli][debug] trio-websocket: 0.11.1
[13:13:40.450332][cli][debug] typing-extensions: 4.9.0
[13:13:40.450742][cli][debug] urllib3: 2.1.0
[13:13:40.451158][cli][debug] websocket-client: 1.7.0
[13:13:40.451318][cli][debug] Arguments:
[13:13:40.451388][cli][debug] url=https://zattoo.com/live/ard
[13:13:40.451450][cli][debug] stream=['worst']
[13:13:40.451516][cli][debug] --loglevel=all
[13:13:40.451574][cli][debug] --logfile=/home/sebastian/tmp/streamlink.log
[13:13:40.451684][cli][debug] --zattoo-email=********
[13:13:40.451743][cli][debug] --zattoo-password=********
[13:13:40.451801][cli][debug] --zattoo-stream-types=['hls7']
[13:13:40.452144][plugins.zattoo][debug] Restored cookies: beaker.session.id, pzuid
[13:13:40.452504][cli][info] Found matching plugin zattoo for URL https://zattoo.com/live/ard
[13:13:40.452598][plugins.zattoo][debug] _watch ...
[13:13:40.452659][plugins.zattoo][debug] get channel ID for ard
[13:13:40.754184][plugins.zattoo][trace] Available zattoo channels in this country: 123tv, 1plus1_ua, 3sat, DE_arte, OstWest, a_tv, al-jazeera, al-jazeera-arabic, allgaeu_tv_de, animal_planet_de, arirang_kr, automotorsporttv, baden_tv_de, baden_tv_sued_de, beauty_tv_de, bein_iz_tr, bein_movies_turk, bibeltv, bloomberg-europe, bloomberg_ht_tr, bloombergoriginals, br, br-alpha, br_nord, cem_tv_tr, channel21, cmc, cnn-international, comedycentral_de, craction_tv_de, curiosity_channel_de, curiosity_now_de, current_time_tv_int, daserste, dazn_fast_de, dazn_rise_de, deluxe-music, deraktionaertv, deutschesmusikfernsehen, df1_de, discovery_de, dmax, dmsat, esports1_de, espreso_tv_ua, euronews-en, euronews-fr, euronews_italian, euronews_russian, euronewsgerman, eurosport1, eurosport2_de, fabella_de, fashiontv, filmgold_de, folx_tv_at, france-24-en, france-24-fr, franken_fernsehen, god-channel-tv, goldstar_tv, grjngo_de, haber_turk, halk_tv, health_tv_de, heimatkanal, heimatkino_de, hgtv_de, hr, hrt1, hrt4, hrt5, hse24, hse24_extra, hse24_trend, itvn, itvn_extra_pl, kabel1_doku, kabel_eins_classics, kabel_eins_deutschland, kanal7avrupa, kika, kinomir, kinowelt, klasik_tv_hr, mdr-sachsen, mdr-sachsen-anhalt, mdr-thueringen, mediaset_italia, meine_einkaufswelt_de, moconomy_de, more_than_sports_tv_de, motorvision_tv, moviedome_de, moviedome_family_de, mtvgermany, muenchen_tv, mytvplus_de, n24_doku, nahe_tv_de, naruto_de, ndr-hamburg, ndr-mv, ndr-niedersachsen, ndr-schleswig-holstein, netzkino_fast_de, nhk_world_jp, nick_cc_plus1, niederbayern_tv_deggendorf_de, niederbayern_tv_landshut_de, niederbayern_tv_passau_de, nrwision, ntv_de, oberpfalz_tv_de, ok4_koblenz_de, ok54_trier_de, ok_kaiserslautern_de, ok_weinstrasse_de, oktv_ludwigshafen_de, oktv_mainz_de, oktv_suedwestpfalz_de, one, one_music_television_de, orf2_europe, phoenix, pink_koncert_rs, pink_serije_rs, pinkextra, pinkfilm, pinkfolk, pinkkids, pinkmusic, pinkplus, pinkreality, pro7_deutschland, pro7_fun, pro7maxx, qs24_ch, qvc2, qvc_germany, qvc_style, radio-bremen-tv, rai-due, rai-tre, rai1_int_it, rainews, rbb, rbb-brandenburg, red_bull_tv_dach_int, regio_tv_schwaben_de, rfo_de, rheinlokal_de, ric, rocketbeans, romance_tv, rtl2_deutschland, rtlDB_geo, rtlDB_rtl_crime, rtlDB_rtl_living, rtlDB_rtl_passion, rtl_bayern_de, rtl_deutschland, rtl_hb_nds_de, rtl_hessen_de, rtl_hh_sh_de, rtl_nrw_de, rtl_ron_de, rtl_up_de, rtlnitro_de, saarland_fernsehen1, sachsen_eins_de, sachsen_fernsehen_chemnitz_de, sachsen_fernsehen_dresden_de, sachsen_fernsehen_leipzig_de, sachsen_fernsehen_vogtland_de, sat1_deutschland, sat1_emotions, sat1gold, schlager_deluxe_de, servus_tv_deutschland, sf-info-intl, showmax_tr, sixx_deutschland, sonlife, sonnenklartv, spiegel_geschichte, spiegel_tv_konflikte_de, sport1, sport1_plus, sportdigital_fussball, sr-fernsehen, starke_frauen_de, stimmungsgarten_tv_at, strongman_champions_league_de, super_rtl_deutschland, swr-fernsehen-bw, swr-fernsehen-rp, sylt_1_de, tagesschau24, tele-5, telebom, tempora_tv_de, terra_mater_wild_de, tlc, toggo_plus, top_filme_de, top_true_crime_de, tr_euro_d, tr_euro_star, tr_show_turk, tv8, tv_berlin_de, tv_ingolstadt_de, tv_mainfranken_de, tv_oberfranken_de, tva_ostbayern_de, tvn24, uatv_ua, vox_deutschland, vox_up_de, warner_tv_comedy, warner_tv_film, warner_tv_serie, wdr-aachen, wdr-bielefeld, wdr-bonn, wdr-dortmund, wdr-duesseldorf, wdr-duisburg, wdr-essen, wdr-koeln, wdr-muenster, wdr-siegen, wdr-wuppertal, wedo_big_stories_de, wedo_movies_de, wedo_sports_de, weihnachtskino_de, welt, weltderwunder, wir_24_tv_de, world_of_freesports_de, zdf, zdf-info, zdfneo, zwei_music_television_de
[13:13:40.754462][plugins.zattoo][debug] CHANNEL ID: ard
[13:13:40.819589][plugins.zattoo][debug] Found data for hls7
[13:13:40.874543][utils.l10n][debug] Language code: de_DE
[13:13:41.089581][stream.hls.m3u8][all] #EXT-X-VERSION:7
[13:13:41.090026][stream.hls.m3u8][all] #EXT-X-INDEPENDENT-SEGMENTS
[13:13:41.090302][stream.hls.m3u8][all] #EXT-X-MEDIA:TYPE=AUDIO,GROUP-ID="audio-mp4a.40.2",NAME="Deutsch",DEFAULT=YES,AUTOSELECT=YES,LANGUAGE="de",URI="t_track_audio_bw_128000_num_0_tid_2_p_10_l_de_nd_1600_mbr_8000.m3u8?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK"
[13:13:41.090803][stream.hls.m3u8][all] #EXT-X-MEDIA:TYPE=AUDIO,GROUP-ID="audio-mp4a.40.2",NAME="Other (mis)",DEFAULT=NO,AUTOSELECT=YES,LANGUAGE="mis",URI="t_track_audio_bw_128000_num_2_tid_4_p_10_l_mis_nd_1600_mbr_8000.m3u8?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK"
[13:13:41.091280][stream.hls.m3u8][all] #EXT-X-MEDIA:TYPE=AUDIO,GROUP-ID="audio-mp4a.40.2",NAME="Other (qks)",DEFAULT=NO,AUTOSELECT=YES,LANGUAGE="qks",URI="t_track_audio_bw_128000_num_4_tid_6_p_10_l_qks_nd_1600_mbr_8000.m3u8?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK"
[13:13:41.091711][stream.hls.m3u8][all] #EXT-X-MEDIA:TYPE=SUBTITLES,GROUP-ID="subs",NAME="Deutsch",LANGUAGE="de",URI="t_track_subs_bw_8000000_num_0_tid_8_l_de_nd_1600_mbr_8000.m3u8?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK"
[13:13:41.092160][stream.hls.m3u8][all] #EXT-X-STREAM-INF:BANDWIDTH=8000000,CODECS="avc1.4d402a,mp4a.40.2",RESOLUTION=1920x1080,FRAME-RATE=50,AUDIO="audio-mp4a.40.2",SUBTITLES="subs",CLOSED-CAPTIONS=NONE
[13:13:41.092510][stream.hls.m3u8][all] t_track_video_bw_7800000_num_0_tid_1_nd_1600_mbr_8000.m3u8?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.092946][stream.hls.m3u8][all] #EXT-X-STREAM-INF:BANDWIDTH=5000000,CODECS="avc1.4d4020,mp4a.40.2",RESOLUTION=1280x720,FRAME-RATE=50,AUDIO="audio-mp4a.40.2",SUBTITLES="subs",CLOSED-CAPTIONS=NONE
[13:13:41.093290][stream.hls.m3u8][all] t_track_video_bw_4800000_num_0_tid_1_nd_1600_mbr_8000.m3u8?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.093680][stream.hls.m3u8][all] #EXT-X-STREAM-INF:BANDWIDTH=3000000,CODECS="avc1.4d401f,mp4a.40.2",RESOLUTION=1280x720,FRAME-RATE=25,AUDIO="audio-mp4a.40.2",SUBTITLES="subs",CLOSED-CAPTIONS=NONE
[13:13:41.094046][stream.hls.m3u8][all] t_track_video_bw_2800000_num_0_tid_1_nd_1600_mbr_8000.m3u8?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.094455][stream.hls.m3u8][all] #EXT-X-STREAM-INF:BANDWIDTH=1500000,CODECS="avc1.4d401e,mp4a.40.2",RESOLUTION=768x432,FRAME-RATE=25,AUDIO="audio-mp4a.40.2",SUBTITLES="subs",CLOSED-CAPTIONS=NONE
[13:13:41.094761][stream.hls.m3u8][all] t_track_video_bw_1300000_num_0_tid_1_nd_1600_mbr_8000.m3u8?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.095156][stream.hls.m3u8][all] #EXT-X-STREAM-INF:BANDWIDTH=900000,CODECS="avc1.4d4015,mp4a.40.2",RESOLUTION=512x288,FRAME-RATE=25,AUDIO="audio-mp4a.40.2",SUBTITLES="subs",CLOSED-CAPTIONS=NONE
[13:13:41.095450][stream.hls.m3u8][all] t_track_video_bw_700000_num_0_tid_1_nd_1600_mbr_8000.m3u8?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.095814][stream.hls.m3u8][all] #EXT-X-STREAM-INF:BANDWIDTH=602000,CODECS="avc1.4d4015,mp4a.40.2",RESOLUTION=512x288,FRAME-RATE=25,AUDIO="audio-mp4a.40.2",SUBTITLES="subs",CLOSED-CAPTIONS=NONE
[13:13:41.096186][stream.hls.m3u8][all] t_track_video_bw_452000_num_0_tid_1_nd_1600_mbr_8000.m3u8?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.097110][stream.ffmpegmux][trace] Querying FFmpeg version: ['/usr/bin/ffmpeg', '-version']
[13:13:41.278374][stream.ffmpegmux][debug] ffmpeg version 6.0.1 Copyright (c) 2000-2023 the FFmpeg developers
[13:13:41.278507][stream.ffmpegmux][debug] built with gcc 12 (Debian 12.2.0-14)
[13:13:41.278572][stream.ffmpegmux][debug] configuration: --disable-decoder=amrnb --disable-decoder=libopenjpeg --disable-gnutls --disable-liblensfun --disable-libopencv --disable-podpages --disable-sndio --disable-stripping --enable-avfilter --enable-chromaprint --enable-frei0r --enable-gcrypt --enable-gpl --enable-ladspa --enable-libaom --enable-libaribb24 --enable-libass --enable-libbluray --enable-libbs2b --enable-libcaca --enable-libcdio --enable-libcodec2 --enable-libdav1d --enable-libdavs2 --enable-libdc1394 --enable-libdrm --enable-libfdk-aac --enable-libflite --enable-libfontconfig --enable-libfreetype --enable-libfribidi --enable-libglslang --enable-libgme --enable-libgsm --enable-libiec61883 --enable-libjack --enable-libkvazaar --enable-libmp3lame --enable-libmysofa --enable-libopencore-amrnb --enable-libopencore-amrwb --enable-libopenh264 --enable-libopenjpeg --enable-libopenmpt --enable-libopus --enable-libpulse --enable-librabbitmq --enable-librist --enable-librsvg --enable-librubberband --enable-libshine --enable-libsmbclient --enable-libsnappy --enable-libsoxr --enable-libspeex --enable-libsrt --enable-libsvtav1 --enable-libtesseract --enable-libtheora --enable-libtwolame --enable-libvidstab --enable-libvo-amrwbenc --enable-libvorbis --enable-libvpx --enable-libwebp --enable-libwebp --enable-libx264 --enable-libx265 --enable-libxavs2 --enable-libxml2 --enable-libxvid --enable-libzimg --enable-libzmq --enable-libzvbi --enable-lv2 --enable-nonfree --enable-omx --enable-openal --enable-opencl --enable-opengl --enable-openssl --enable-postproc --enable-pthreads --enable-shared --enable-version3 --enable-vulkan --incdir=/usr/include/x86_64-linux-gnu --libdir=/usr/lib/x86_64-linux-gnu --prefix=/usr --toolchain=hardened --enable-vaapi --enable-libvpl --enable-libvmaf --enable-libilbc --enable-libjxl --cc=x86_64-linux-gnu-gcc --cxx=x86_64-linux-gnu-g++ --disable-altivec --shlibdir=/usr/lib/x86_64-linux-gnu
[13:13:41.278632][stream.ffmpegmux][debug] libavutil 58. 2.100 / 58. 2.100
[13:13:41.278687][stream.ffmpegmux][debug] libavcodec 60. 3.100 / 60. 3.100
[13:13:41.278759][stream.ffmpegmux][debug] libavformat 60. 3.100 / 60. 3.100
[13:13:41.278813][stream.ffmpegmux][debug] libavdevice 60. 1.100 / 60. 1.100
[13:13:41.278867][stream.ffmpegmux][debug] libavfilter 9. 3.100 / 9. 3.100
[13:13:41.278919][stream.ffmpegmux][debug] libswscale 7. 1.100 / 7. 1.100
[13:13:41.278971][stream.ffmpegmux][debug] libswresample 4. 10.100 / 4. 10.100
[13:13:41.279022][stream.ffmpegmux][debug] libpostproc 57. 1.100 / 57. 1.100
[13:13:41.279089][stream.hls][debug] Using external audio tracks for stream 1080p (language=de, name=Deutsch)
[13:13:41.279232][stream.hls][debug] Using external audio tracks for stream 720p (language=de, name=Deutsch)
[13:13:41.279346][stream.hls][debug] Using external audio tracks for stream 720p_alt (language=de, name=Deutsch)
[13:13:41.279452][stream.hls][debug] Using external audio tracks for stream 432p (language=de, name=Deutsch)
[13:13:41.279558][stream.hls][debug] Using external audio tracks for stream 288p (language=de, name=Deutsch)
[13:13:41.279660][stream.hls][debug] Using external audio tracks for stream 288p_alt (language=de, name=Deutsch)
[13:13:41.279829][utils.l10n][debug] Language code: de_DE
[13:13:41.314935][stream.hls.m3u8][all] #EXT-X-VERSION:7
[13:13:41.315129][stream.hls.m3u8][all] #EXT-X-INDEPENDENT-SEGMENTS
[13:13:41.315215][stream.hls.m3u8][all] #EXT-X-MEDIA:TYPE=AUDIO,GROUP-ID="audio-mp4a.40.2",NAME="Deutsch",DEFAULT=YES,AUTOSELECT=YES,LANGUAGE="de",URI="t_track_audio_bw_128000_num_0_tid_2_p_10_l_de_nd_1600_mbr_602.m3u8?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK"
[13:13:41.315370][stream.hls.m3u8][all] #EXT-X-MEDIA:TYPE=AUDIO,GROUP-ID="audio-mp4a.40.2",NAME="Other (mis)",DEFAULT=NO,AUTOSELECT=YES,LANGUAGE="mis",URI="t_track_audio_bw_128000_num_2_tid_4_p_10_l_mis_nd_1600_mbr_602.m3u8?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK"
[13:13:41.315511][stream.hls.m3u8][all] #EXT-X-MEDIA:TYPE=AUDIO,GROUP-ID="audio-mp4a.40.2",NAME="Other (qks)",DEFAULT=NO,AUTOSELECT=YES,LANGUAGE="qks",URI="t_track_audio_bw_128000_num_4_tid_6_p_10_l_qks_nd_1600_mbr_602.m3u8?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK"
[13:13:41.315638][stream.hls.m3u8][all] #EXT-X-MEDIA:TYPE=SUBTITLES,GROUP-ID="subs",NAME="Deutsch",LANGUAGE="de",URI="t_track_subs_bw_602000_num_0_tid_8_l_de_nd_1600_mbr_602.m3u8?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK"
[13:13:41.315770][stream.hls.m3u8][all] #EXT-X-STREAM-INF:BANDWIDTH=602000,CODECS="avc1.4d4015,mp4a.40.2",RESOLUTION=512x288,FRAME-RATE=25,AUDIO="audio-mp4a.40.2",SUBTITLES="subs",CLOSED-CAPTIONS=NONE
[13:13:41.315864][stream.hls.m3u8][all] t_track_video_bw_452000_num_0_tid_1_nd_1600_mbr_602.m3u8?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.316089][stream.hls][debug] Using external audio tracks for stream 288p (language=de, name=Deutsch)
[13:13:41.316932][cli][info] Available streams: 288p_alt2 (worst), 288p_alt, 288p, 432p, 720p_alt, 720p, 1080p (best)
[13:13:41.317072][cli][info] Opening stream: 288p_alt2 (hls-multi)
[13:13:41.317172][cli][info] Starting player: /usr/bin/vlc
[13:13:41.317298][stream.ffmpegmux][debug] Opening hls substream
[13:13:41.318360][stream.hls][debug] Reloading playlist
[13:13:41.320342][stream.ffmpegmux][debug] Opening hls substream
[13:13:41.321462][stream.hls][debug] Reloading playlist
[13:13:41.323576][utils.named_pipe][info] Creating pipe streamlinkpipe-27121-1-1196
[13:13:41.324001][utils.named_pipe][info] Creating pipe streamlinkpipe-27121-2-175
[13:13:41.324243][stream.ffmpegmux][debug] ffmpeg command: /usr/bin/ffmpeg -nostats -y -i /tmp/streamlinkpipe-27121-1-1196 -i /tmp/streamlinkpipe-27121-2-175 -c:v copy -c:a copy -map 0:v? -map 0:a? -map 1:a -f mpegts pipe:1
[13:13:41.324566][stream.ffmpegmux][debug] Starting copy to pipe: /tmp/streamlinkpipe-27121-1-1196
[13:13:41.324951][stream.ffmpegmux][debug] Starting copy to pipe: /tmp/streamlinkpipe-27121-2-175
[13:13:41.325609][cli][debug] Pre-buffering 8192 bytes
[13:13:41.351448][stream.hls.m3u8][all] #EXT-X-VERSION:7
[13:13:41.351679][stream.hls.m3u8][all] #EXT-X-TARGETDURATION:2
[13:13:41.351815][stream.hls.m3u8][all] #EXT-X-MEDIA-SEQUENCE:1064637460
[13:13:41.351934][stream.hls.m3u8][all] #EXT-X-PROGRAM-DATE-TIME:2023-12-24T12:12:16.000+00:00
[13:13:41.357157][stream.hls.m3u8][all] #EXT-X-MAP:URI="i_track_video_bw_452000_nd_1600_num_0_tid_1_mbr_602.mp4?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK"
[13:13:41.357453][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.357633][stream.hls.m3u8][all] f_track_video_ts_1703419936000_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.357840][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.357986][stream.hls.m3u8][all] f_track_video_ts_1703419937600_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.358183][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.358326][stream.hls.m3u8][all] f_track_video_ts_1703419939200_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.358522][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.358644][stream.hls.m3u8][all] f_track_video_ts_1703419940800_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.358859][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.359003][stream.hls.m3u8][all] f_track_video_ts_1703419942400_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.359172][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.359417][stream.hls.m3u8][all] f_track_video_ts_1703419944000_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.359592][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.359730][stream.hls.m3u8][all] f_track_video_ts_1703419945600_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.359881][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.360001][stream.hls.m3u8][all] f_track_video_ts_1703419947200_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.360150][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.360276][stream.hls.m3u8][all] f_track_video_ts_1703419948800_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.360447][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.360600][stream.hls.m3u8][all] f_track_video_ts_1703419950400_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.360754][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.360881][stream.hls.m3u8][all] f_track_video_ts_1703419952000_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.361027][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.361138][stream.hls.m3u8][all] f_track_video_ts_1703419953600_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.361284][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.361408][stream.hls.m3u8][all] f_track_video_ts_1703419955200_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.361551][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.361665][stream.hls.m3u8][all] f_track_video_ts_1703419956800_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.361802][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.361917][stream.hls.m3u8][all] f_track_video_ts_1703419958400_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.362057][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.362168][stream.hls.m3u8][all] f_track_video_ts_1703419960000_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.362303][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.362416][stream.hls.m3u8][all] f_track_video_ts_1703419961600_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.362563][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.362671][stream.hls.m3u8][all] f_track_video_ts_1703419963200_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.362806][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.362924][stream.hls.m3u8][all] f_track_video_ts_1703419964800_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.363065][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.363177][stream.hls.m3u8][all] f_track_video_ts_1703419966400_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.363319][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.363691][stream.hls.m3u8][all] f_track_video_ts_1703419968000_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.363849][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.363983][stream.hls.m3u8][all] f_track_video_ts_1703419969600_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.364130][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.364250][stream.hls.m3u8][all] f_track_video_ts_1703419971200_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.364394][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.364522][stream.hls.m3u8][all] f_track_video_ts_1703419972800_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.364662][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.364777][stream.hls.m3u8][all] f_track_video_ts_1703419974400_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.364922][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.365032][stream.hls.m3u8][all] f_track_video_ts_1703419976000_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.365172][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.365289][stream.hls.m3u8][all] f_track_video_ts_1703419977600_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.365438][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.365554][stream.hls.m3u8][all] f_track_video_ts_1703419979200_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.365697][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.365820][stream.hls.m3u8][all] f_track_video_ts_1703419980800_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.365965][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.366079][stream.hls.m3u8][all] f_track_video_ts_1703419982400_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.366224][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.366360][stream.hls.m3u8][all] f_track_video_ts_1703419984000_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.366511][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.366627][stream.hls.m3u8][all] f_track_video_ts_1703419985600_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.366792][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.366911][stream.hls.m3u8][all] f_track_video_ts_1703419987200_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.367062][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.367206][stream.hls.m3u8][all] f_track_video_ts_1703419988800_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.367367][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.367483][stream.hls.m3u8][all] f_track_video_ts_1703419990400_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.367625][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.367753][stream.hls.m3u8][all] f_track_video_ts_1703419992000_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.367912][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.368026][stream.hls.m3u8][all] f_track_video_ts_1703419993600_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.368171][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.368295][stream.hls.m3u8][all] f_track_video_ts_1703419995200_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.368456][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.368572][stream.hls.m3u8][all] f_track_video_ts_1703419996800_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.368715][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.368838][stream.hls.m3u8][all] f_track_video_ts_1703419998400_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.368983][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.369099][stream.hls.m3u8][all] f_track_video_ts_1703420000000_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.369245][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.369374][stream.hls.m3u8][all] f_track_video_ts_1703420001600_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.369530][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.369649][stream.hls.m3u8][all] f_track_video_ts_1703420003200_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.369806][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.369942][stream.hls.m3u8][all] f_track_video_ts_1703420004800_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.370118][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.370240][stream.hls.m3u8][all] f_track_video_ts_1703420006400_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.370391][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.370519][stream.hls.m3u8][all] f_track_video_ts_1703420008000_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.370699][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.370821][stream.hls.m3u8][all] f_track_video_ts_1703420009600_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.370969][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.371096][stream.hls.m3u8][all] f_track_video_ts_1703420011200_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.371245][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.371360][stream.hls.m3u8][all] f_track_video_ts_1703420012800_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.371509][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.371630][stream.hls.m3u8][all] f_track_video_ts_1703420014400_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.371877][stream.hls][debug] First Sequence: 1064637460; Last Sequence: 1064637509
[13:13:41.372004][stream.hls][debug] Start offset: 0; Duration: None; Start Sequence: 1064637507; End Sequence: None
[13:13:41.372134][stream.hls][debug] Adding segment 1064637507 to queue
[13:13:41.374210][stream.hls][debug] Adding segment 1064637508 to queue
[13:13:41.375094][stream.hls][debug] Adding segment 1064637509 to queue
[13:13:41.471166][stream.hls][debug] Writing segment 1064637507 to output
[13:13:41.472345][stream.hls][debug] Segment initialization 1064637507 complete
[13:13:41.545802][stream.hls.m3u8][all] #EXT-X-VERSION:7
[13:13:41.545988][stream.hls.m3u8][all] #EXT-X-TARGETDURATION:2
[13:13:41.546077][stream.hls.m3u8][all] #EXT-X-MEDIA-SEQUENCE:1064637461
[13:13:41.546155][stream.hls.m3u8][all] #EXT-X-PROGRAM-DATE-TIME:2023-12-24T12:12:17.600+00:00
[13:13:41.546290][stream.hls.m3u8][all] #EXT-X-MAP:URI="i_track_audio_bw_128000_nd_1600_num_0_tid_2_mbr_602_p_10.mp4?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK"
[13:13:41.546428][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.546525][stream.hls.m3u8][all] f_track_audio_ts_1703419937600_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.546638][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.546722][stream.hls.m3u8][all] f_track_audio_ts_1703419939200_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.546829][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.546920][stream.hls.m3u8][all] f_track_audio_ts_1703419940800_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.547026][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.547109][stream.hls.m3u8][all] f_track_audio_ts_1703419942400_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.547234][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.547327][stream.hls.m3u8][all] f_track_audio_ts_1703419944000_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.547432][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.547513][stream.hls.m3u8][all] f_track_audio_ts_1703419945600_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.547615][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.547702][stream.hls.m3u8][all] f_track_audio_ts_1703419947200_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.547804][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.547884][stream.hls.m3u8][all] f_track_audio_ts_1703419948800_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.547984][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.548080][stream.hls.m3u8][all] f_track_audio_ts_1703419950400_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.548228][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.548347][stream.hls.m3u8][all] f_track_audio_ts_1703419952000_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.548467][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.548549][stream.hls.m3u8][all] f_track_audio_ts_1703419953600_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.548647][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.548732][stream.hls.m3u8][all] f_track_audio_ts_1703419955200_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.548831][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.548908][stream.hls.m3u8][all] f_track_audio_ts_1703419956800_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.549010][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.549094][stream.hls.m3u8][all] f_track_audio_ts_1703419958400_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.549191][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.549269][stream.hls.m3u8][all] f_track_audio_ts_1703419960000_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.549366][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.549450][stream.hls.m3u8][all] f_track_audio_ts_1703419961600_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.549564][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.549643][stream.hls.m3u8][all] f_track_audio_ts_1703419963200_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.549740][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.549823][stream.hls.m3u8][all] f_track_audio_ts_1703419964800_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.549920][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.550012][stream.hls.m3u8][all] f_track_audio_ts_1703419966400_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.550121][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.550215][stream.hls.m3u8][all] f_track_audio_ts_1703419968000_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.550327][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.550413][stream.hls.m3u8][all] f_track_audio_ts_1703419969600_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.550523][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.550617][stream.hls.m3u8][all] f_track_audio_ts_1703419971200_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.550727][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.550814][stream.hls.m3u8][all] f_track_audio_ts_1703419972800_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.550927][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.551022][stream.hls.m3u8][all] f_track_audio_ts_1703419974400_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.551133][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.551219][stream.hls.m3u8][all] f_track_audio_ts_1703419976000_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.551370][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.551500][stream.hls.m3u8][all] f_track_audio_ts_1703419977600_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.551621][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.551710][stream.hls.m3u8][all] f_track_audio_ts_1703419979200_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.551828][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.551937][stream.hls.m3u8][all] f_track_audio_ts_1703419980800_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.552051][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.552145][stream.hls.m3u8][all] f_track_audio_ts_1703419982400_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.552257][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.552344][stream.hls.m3u8][all] f_track_audio_ts_1703419984000_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.552471][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.552569][stream.hls.m3u8][all] f_track_audio_ts_1703419985600_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.552682][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.552770][stream.hls.m3u8][all] f_track_audio_ts_1703419987200_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.552880][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.552976][stream.hls.m3u8][all] f_track_audio_ts_1703419988800_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.553093][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.553181][stream.hls.m3u8][all] f_track_audio_ts_1703419990400_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.553289][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.553382][stream.hls.m3u8][all] f_track_audio_ts_1703419992000_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.553492][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.553580][stream.hls.m3u8][all] f_track_audio_ts_1703419993600_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.553690][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.553796][stream.hls.m3u8][all] f_track_audio_ts_1703419995200_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.553946][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.554067][stream.hls.m3u8][all] f_track_audio_ts_1703419996800_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.554186][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.554282][stream.hls.m3u8][all] f_track_audio_ts_1703419998400_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.554410][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.554501][stream.hls.m3u8][all] f_track_audio_ts_1703420000000_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.554611][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.554707][stream.hls.m3u8][all] f_track_audio_ts_1703420001600_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.554818][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.554905][stream.hls.m3u8][all] f_track_audio_ts_1703420003200_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.555035][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.555143][stream.hls.m3u8][all] f_track_audio_ts_1703420004800_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.555272][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.555374][stream.hls.m3u8][all] f_track_audio_ts_1703420006400_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.555501][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.555610][stream.hls.m3u8][all] f_track_audio_ts_1703420008000_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.555739][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.555839][stream.hls.m3u8][all] f_track_audio_ts_1703420009600_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.556005][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.556106][stream.hls.m3u8][all] f_track_audio_ts_1703420011200_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.556233][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.556343][stream.hls.m3u8][all] f_track_audio_ts_1703420012800_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.556489][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.556596][stream.hls.m3u8][all] f_track_audio_ts_1703420014400_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.556721][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:41.556828][stream.hls.m3u8][all] f_track_audio_ts_1703420016000_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:41.557026][stream.hls][debug] First Sequence: 1064637461; Last Sequence: 1064637510
[13:13:41.557139][stream.hls][debug] Start offset: 0; Duration: None; Start Sequence: 1064637508; End Sequence: None
[13:13:41.557259][stream.hls][debug] Adding segment 1064637508 to queue
[13:13:41.559625][stream.hls][debug] Adding segment 1064637509 to queue
[13:13:41.559848][stream.hls][debug] Adding segment 1064637510 to queue
[13:13:41.705513][stream.hls][debug] Writing segment 1064637507 to output
[13:13:41.706574][stream.hls][debug] Segment 1064637507 complete
[13:13:41.712411][stream.hls][debug] Writing segment 1064637508 to output
[13:13:41.713772][stream.hls][debug] Segment initialization 1064637508 complete
[13:13:41.935191][stream.hls][debug] Writing segment 1064637508 to output
[13:13:41.936157][stream.hls][debug] Segment 1064637508 complete
[13:13:42.075059][stream.hls][debug] Writing segment 1064637508 to output
[13:13:42.075694][stream.hls][debug] Segment 1064637508 complete
[13:13:42.103842][cli.output][debug] Opening subprocess: ['/usr/bin/vlc', '--input-title-format', 'https://zattoo.com/live/ard', '-']
[13:13:42.200054][stream.hls][debug] Writing segment 1064637509 to output
[13:13:42.200351][stream.hls][debug] Segment 1064637509 complete
[13:13:42.242108][stream.hls][debug] Writing segment 1064637509 to output
[13:13:42.242329][stream.hls][debug] Segment 1064637509 complete
[13:13:42.355326][stream.hls][debug] Writing segment 1064637510 to output
[13:13:42.355581][stream.hls][debug] Segment 1064637510 complete
[13:13:42.606609][cli][debug] Writing stream to output
[13:13:43.319077][stream.hls][debug] Reloading playlist
[13:13:43.324662][stream.hls][debug] Reloading playlist
[13:13:43.364717][stream.hls.m3u8][all] #EXT-X-VERSION:7
[13:13:43.365288][stream.hls.m3u8][all] #EXT-X-TARGETDURATION:2
[13:13:43.365579][stream.hls.m3u8][all] #EXT-X-MEDIA-SEQUENCE:1064637462
[13:13:43.365834][stream.hls.m3u8][all] #EXT-X-PROGRAM-DATE-TIME:2023-12-24T12:12:19.200+00:00
[13:13:43.366205][stream.hls.m3u8][all] #EXT-X-MAP:URI="i_track_audio_bw_128000_nd_1600_num_0_tid_2_mbr_602_p_10.mp4?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK"
[13:13:43.366601][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:43.366915][stream.hls.m3u8][all] f_track_audio_ts_1703419939200_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:43.367244][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:43.367510][stream.hls.m3u8][all] f_track_audio_ts_1703419940800_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:43.367813][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:43.368109][stream.hls.m3u8][all] f_track_audio_ts_1703419942400_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:43.368508][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:43.368923][stream.hls.m3u8][all] f_track_audio_ts_1703419944000_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:43.371092][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:43.371824][stream.hls.m3u8][all] f_track_audio_ts_1703419945600_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:43.372215][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:43.372550][stream.hls.m3u8][all] f_track_audio_ts_1703419947200_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:43.373290][stream.hls.m3u8][all] #EXT-X-VERSION:7
[13:13:43.373594][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:43.374074][stream.hls.m3u8][all] #EXT-X-TARGETDURATION:2
[13:13:43.374596][stream.hls.m3u8][all] f_track_audio_ts_1703419948800_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:43.375075][stream.hls.m3u8][all] #EXT-X-MEDIA-SEQUENCE:1064637462
[13:13:43.375695][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:43.376171][stream.hls.m3u8][all] #EXT-X-PROGRAM-DATE-TIME:2023-12-24T12:12:19.200+00:00
[13:13:43.376703][stream.hls.m3u8][all] f_track_audio_ts_1703419950400_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:43.377309][stream.hls.m3u8][all] #EXT-X-MAP:URI="i_track_video_bw_452000_nd_1600_num_0_tid_1_mbr_602.mp4?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK"
[13:13:43.377859][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:43.378446][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:43.378963][stream.hls.m3u8][all] f_track_audio_ts_1703419952000_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:43.379476][stream.hls.m3u8][all] f_track_video_ts_1703419939200_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:43.380638][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:43.380025][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:43.380960][stream.hls.m3u8][all] f_track_video_ts_1703419940800_bw_452000_nd_1600_d_1600_num_0_tid_1_mbr_602.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:43.381417][stream.hls.m3u8][all] f_track_audio_ts_1703419953600_bw_128000_nd_1600_d_1600_num_0_tid_2_mbr_602_p_10_l_de.m4s?z32=MNZWSZB5GE3UCM2DGM3UERCBHBCTSQJXIQWTKN2FIE4DIQJZIRAUGQSGGY2UEJTVONSXEX3JMQ6TGMRWGEYTAOJSEZ3D2MBGONUWOPJYL5SWCMJUGE2DSOJXGAZTAZLGGU4GKMZWMU3DOZDDGE4DGMRUMM3DK
[13:13:43.382033][stream.hls.m3u8][all] #EXTINF:1.600000,
[13:13:43.382599][stream.hls.m3u8][all] #EXTINF:1.600000,
...
```
| > maybe one segment length
> video playlist: `#EXT-X-MEDIA-SEQUENCE:1064637460`
> audio playlist: `#EXT-X-MEDIA-SEQUENCE:1064637461`
https://datatracker.ietf.org/doc/html/rfc8216#section-4.3.3.2
> [4.3.3.2](https://datatracker.ietf.org/doc/html/rfc8216#section-4.3.3.2). EXT-X-MEDIA-SEQUENCE
> A client MUST NOT assume that segments with the same Media Sequence Number in different Media Playlists contain matching content (see [Section 6.3.2](https://datatracker.ietf.org/doc/html/rfc8216#section-6.3.2)).
https://datatracker.ietf.org/doc/html/rfc8216#section-6.3.2
> A client MUST NOT assume that segments with the same Media Sequence Number in different Variant Streams or Renditions have the same position in the presentation; Playlists MAY have independent Media Sequence Numbers. Instead, a client MUST use the relative position of each segment on the Playlist timeline and its Discontinuity Sequence Number to locate corresponding segments.
Both independent transport streams are fed into FFmpeg which then muxes them into one output stream with respect to each presentation time.
Does [`--ffmpeg-copyts`](https://streamlink.github.io/cli.html#cmdoption-ffmpeg-copyts) fix the issue? This option can also be forced by the plugin, so it doesn't have to be set by the user on the CLI.
----
> Opening stream: 288p_alt2
This is a stream of the second multivariant playlist which the plugin yields. Do streams of the main one have the same issue?
> Does [`--ffmpeg-copyts`](https://streamlink.github.io/cli.html#cmdoption-ffmpeg-copyts) fix the issue? This option can also be forced by the plugin, so it doesn't have to be set by the user on the CLI.
Yes, this seems to fix the issue. At least I could not reproduce the problem in a series of about 100 attempts.
>
> > Opening stream: 288p_alt2
>
> This is a stream of the second multivariant playlist which the plugin yields. Do streams of the main one have the same issue?
I tried the 288p and 1080p streams (without the --fmpeg-copyts option) and yes, they are also subject to this issue. | 2023-12-25T09:26:06 |
|
streamlink/streamlink | 5,742 | streamlink__streamlink-5742 | [
"5694"
] | 1d2390ebf757eb714202a2ba03e50566b7e62576 | diff --git a/src/streamlink/plugins/atresplayer.py b/src/streamlink/plugins/atresplayer.py
--- a/src/streamlink/plugins/atresplayer.py
+++ b/src/streamlink/plugins/atresplayer.py
@@ -23,7 +23,7 @@
))
class AtresPlayer(Plugin):
_channels_api_url = "https://api.atresplayer.com/client/v1/info/channels"
- _player_api_url = "https://api.atresplayer.com/player/v1/live/{channel_id}"
+ _player_api_url = "https://api.atresplayer.com/player/v1/live/{channel_id}?NODRM=true"
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
@@ -54,7 +54,7 @@ def _get_streams(self):
"error_description": str,
},
{
- "sources": [
+ "sourcesLive": [
validate.all(
{
"src": validate.url(),
@@ -70,7 +70,7 @@ def _get_streams(self):
log.error(f"Player API error: {sources['error']} - {sources['error_description']}")
return
- for streamtype, streamsrc in sources.get("sources"):
+ for streamtype, streamsrc in sources.get("sourcesLive"):
log.debug(f"Stream source: {streamsrc} ({streamtype or 'n/a'})")
if streamtype == "application/vnd.apple.mpegurl":
| plugins.atresplayer: Error -3 while decompressing data: incorrect header check
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
streamlink 6.4.2
### Description
Possible change in link decoding.
### Debug log
```text
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.11.6
[cli][debug] OpenSSL: OpenSSL 3.0.11 19 Sep 2023
[cli][debug] Streamlink: 6.4.2
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.11.17
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.3
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.19.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.23.1
[cli][debug] trio-websocket: 0.11.1
[cli][debug] typing-extensions: 4.8.0
[cli][debug] urllib3: 2.1.0
[cli][debug] websocket-client: 1.6.4
[cli][debug] Arguments:
[cli][debug] url=https://www.atresplayer.com/directos/antena3/
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --ffmpeg-ffmpeg=C:\Program Files\Streamlink\ffmpeg\ffmpeg.exe
[cli][info] Found matching plugin atresplayer for URL https://www.atresplayer.com/directos/antena3/
[plugins.atresplayer][debug] Player API URL: https://api.atresplayer.com/player/v1/live/5a6a165a7ed1a834493ebf6a
[plugins.atresplayer][debug] Stream source: https://directo.atresmedia.com/49aa0979c14a4113668984aa8f6f7a43dd3a624a_1701338572/antena3/master.m3u8 (application/vnd.apple.mpegurl)
[utils.l10n][debug] Language code: es_ES
error: Unable to open URL: https://directo.atresmedia.com/49aa0979c14a4113668984aa8f6f7a43dd3a624a_1701338572/antena3/master.m3u8 (('Received response with content-encoding: gzip, but failed to decode it.', error('Error -3 while decompressing data: incorrect header check')))
```
| This looks like a server issue/misconfiguration where they send garbage data to the client after it has requested the response to be compressed using `gzip, deflate`. It's possible that this is a temporary issue on their end.
Please apply the following diff and see if it works. The site is geo-blocked and they detect all VPNs I have access to, so I can't test it myself. This change disables compressed HTTP responses (`Accept-Encoding: identity`), but it's possible that their servers ignore this request header as well.
```diff
diff --git a/src/streamlink/plugins/atresplayer.py b/src/streamlink/plugins/atresplayer.py
index 375cc6eb..70b40349 100644
--- a/src/streamlink/plugins/atresplayer.py
+++ b/src/streamlink/plugins/atresplayer.py
@@ -70,17 +70,18 @@ class AtresPlayer(Plugin):
log.error(f"Player API error: {sources['error']} - {sources['error_description']}")
return
+ headers = {"Accept-Encoding": "identity"}
for streamtype, streamsrc in sources.get("sources"):
log.debug(f"Stream source: {streamsrc} ({streamtype or 'n/a'})")
if streamtype == "application/vnd.apple.mpegurl":
- streams = HLSStream.parse_variant_playlist(self.session, streamsrc)
+ streams = HLSStream.parse_variant_playlist(self.session, streamsrc, headers=headers)
if not streams:
- yield "live", HLSStream(self.session, streamsrc)
+ yield "live", HLSStream(self.session, streamsrc, headers=headers)
else:
yield from streams.items()
elif streamtype == "application/dash+xml":
- yield from DASHStream.parse_manifest(self.session, streamsrc).items()
+ yield from DASHStream.parse_manifest(self.session, streamsrc, headers=headers).items()
__plugin__ = AtresPlayer
```
Alternatively, if you don't know how to apply the diff, here's the full plugin file for you to [sideload](https://streamlink.github.io/cli/plugin-sideloading.html):
https://raw.githubusercontent.com/bastimeyer/streamlink/18f8b966622382504e670100a262adf55bb3da45/src/streamlink/plugins/atresplayer.py
Changed the plugin file.
I think there is something else.
```
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.11.6
[cli][debug] OpenSSL: OpenSSL 3.0.11 19 Sep 2023
[cli][debug] Streamlink: 6.4.2
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.11.17
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.3
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.19.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.23.1
[cli][debug] trio-websocket: 0.11.1
[cli][debug] typing-extensions: 4.8.0
[cli][debug] urllib3: 2.1.0
[cli][debug] websocket-client: 1.6.4
[cli][debug] Arguments:
[cli][debug] url=https://www.atresplayer.com/directos/antena3/
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --ffmpeg-ffmpeg=C:\Program Files\Streamlink\ffmpeg\ffmpeg.exe
[cli][info] Found matching plugin atresplayer for URL https://www.atresplayer.com/directos/antena3/
[plugins.atresplayer][debug] Player API URL: https://api.atresplayer.com/player/v1/live/5a6a165a7ed1a834493ebf6a
[plugins.atresplayer][debug] Stream source: https://directo.atresmedia.com/0883d6c329e97c8f19dd5a1a9580fff6885726bb_1701341548/antena3/master.m3u8 (application/vnd.apple.mpegurl)
[utils.l10n][debug] Language code: es_ES
error: Unable to open URL: https://directo.atresmedia.com/0883d6c329e97c8f19dd5a1a9580fff6885726bb_1701341548/antena3/master.m3u8 (404 Client Error: Not Found for url: https://directo.atresmedia.com/0883d6c329e97c8f19dd5a1a9580fff6885726bb_1701341548/antena3/master.m3u8)
```
> 404 Client Error
That doesn't seem right. Just to be sure that you didn't mess up the plugin changes via the diff, please instead sideload the plugin file I've linked. If done correctly, there should be a debug log message at the top.
However, in case they indeed return 404 with the `Accept-Encoding: identity` header being set, then my only other idea would be installing the `brotli` and/or `zstandard` packages in Streamlink's Python environment, so `urllib3` can pick these other compression algorithms up automatically. Since you appear to be using Streamlink's Windows builds though, you'll either have to install Streamlink in a local Python environment on your own, so you can use pip and install the dependencies, or you'll have to wait for me until tomorrow, so I can add them to Streamlink's Windows builds (which is a good idea anyway).
I can confirm the 404 Client Error with the plugin change and/or header.
In fact it's the exact same situation we talked one year ago there: https://github.com/streamlink/streamlink/issues/4895
https://github.com/streamlink/streamlink/issues/4895#issuecomment-1288053745
> The old m3u8 that was down these last days started to work again today. Let's be attentive and see if it's temporary or find a solution. On the website it still load the new url.
----
If you want this to get fixed, so the plugin uses the same stream URLs as their website, then please open a pull request with the necessary changes. Nobody with access to the API has shown interest to fix this yet. However, there was a recent plugin change in August via #5477.
Hi, there is an issue with this plugin. Hope you'll be able to fix it soon.
Hello,
Can you please test this? I think this modification works.
[atresplayer_dec2023.zip](https://github.com/streamlink/streamlink/files/13692572/atresplayer_dec2023.zip)
Best regards.
----
edit (added diff for transparency reasons)
```diff
diff --git a/src/streamlink/plugins/atresplayer.py b/tmp/.psub.DMeopkXmHb
index 375cc6e..a0ca632 100644
--- a/src/streamlink/plugins/atresplayer.py
+++ b/tmp/.psub.DMeopkXmHb
@@ -23,7 +23,7 @@ log = logging.getLogger(__name__)
))
class AtresPlayer(Plugin):
_channels_api_url = "https://api.atresplayer.com/client/v1/info/channels"
- _player_api_url = "https://api.atresplayer.com/player/v1/live/{channel_id}"
+ _player_api_url = "https://api.atresplayer.com/player/v1/live/{channel_id}?NODRM=true"
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
@@ -54,7 +54,7 @@ class AtresPlayer(Plugin):
"error_description": str,
},
{
- "sources": [
+ "sourcesLive": [
validate.all(
{
"src": validate.url(),
@@ -70,7 +70,7 @@ class AtresPlayer(Plugin):
log.error(f"Player API error: {sources['error']} - {sources['error_description']}")
return
- for streamtype, streamsrc in sources.get("sources"):
+ for streamtype, streamsrc in sources.get("sourcesLive"):
log.debug(f"Stream source: {streamsrc} ({streamtype or 'n/a'})")
if streamtype == "application/vnd.apple.mpegurl":
```
After replace atresplayer.py it does not work, sorry.
This the log . (Sorry, i do not know, how to format correctly)
Dec 19 17:42:42 ubuntu tvheadend[65096]: spawn: Executing "/home/hts/.local/bin/streamlink"
Dec 19 17:42:44 ubuntu tvheadend[65096]: spawn: [cli][info] Found matching plugin atresplayer for URL https://www.atresplayer.com/directos/antena3/
Dec 19 17:42:44 ubuntu tvheadend[65096]: spawn: [cli][info] Available streams: 360p (worst), 480p, 720p, 1080p (best)
Dec 19 17:42:44 ubuntu tvheadend[65096]: spawn: [cli][info] Opening stream: 1080p (hls)
Dec 19 17:42:47 ubuntu tvheadend[65096]: spawn: [stream.hls][error] Failed to fetch segment 170121195: Unable to open URL: https://directo.atresmedia.com/147a6ebb30b6857da59de163cee5aff8cfbbc6a7_1703032964/geoantena3/2/br4/230619065411/94511/br4_170121195.ts (404 Client Error: Not Found for url: https://directo.atresmedia.com/147a6ebb30b6857da59de163cee5aff8cfbbc6a7_1703032964/geoantena3/2/br4/230619065411/94511/br4_170121195.ts)
Dec 19 17:42:49 ubuntu tvheadend[65096]: spawn: [stream.hls][error] Failed to fetch segment 170121196: Unable to open URL: https://directo.atresmedia.com/147a6ebb30b6857da59de163cee5aff8cfbbc6a7_1703032964/geoantena3/2/br4/230619065411/94511/br4_170121196.ts (404 Client Error: Not Found for url: https://directo.atresmedia.com/147a6ebb30b6857da59de163cee5aff8cfbbc6a7_1703032964/geoantena3/2/br4/230619065411/94511/br4_170121196.ts)
Dec 19 17:42:51 ubuntu tvheadend[65096]: spawn: [stream.hls][error] Failed to fetch segment 170121197: Unable to open URL: https://directo.atresmedia.com/147a6ebb30b6857da59de163cee5aff8cfbbc6a7_1703032964/geoantena3/2/br4/230619065411/94511/br4_170121197.ts (404 Client Error: Not Found for url: https://directo.atresmedia.com/147a6ebb30b6857da59de163cee5aff8cfbbc6a7_1703032964/geoantena3/2/br4/230619065411/94511/br4_170121197.ts)
Don't know if you're testing in Spain or abroad. I'm using streamlink 6.4.2-1 on Windows and the modification I proposed works like a charm!
streamlink atresplayer.com/directos/neox best
[cli][info] Found matching plugin atresplayer for URL atresplayer.com/directos/neox
[cli][info] Available streams: 360p (worst), 480p, 720p, 1080p (best)
[cli][info] Opening stream: 1080p (hls-multi)
[cli][info] Starting player: C:\Program Files\VideoLAN\VLC\vlc.exe
[utils.named_pipe][info] Creating pipe streamlinkpipe-17936-1-4814
[utils.named_pipe][info] Creating pipe streamlinkpipe-17936-2-6528
If you're sure that your changes do indeed fix the plugin, then please submit a pull request with a full debug log running Streamlink from your PR branch, so we can verify that it's working. Ideally with debug logs from multiple channels. Thanks.
I confirm that this changes work on 6.5.0 on Linux.
Works great, even though have delay in some channels.
Works fine for me | 2023-12-29T09:54:00 |
|
streamlink/streamlink | 5,745 | streamlink__streamlink-5745 | [
"5744"
] | 1d2390ebf757eb714202a2ba03e50566b7e62576 | diff --git a/src/streamlink/plugins/huya.py b/src/streamlink/plugins/huya.py
--- a/src/streamlink/plugins/huya.py
+++ b/src/streamlink/plugins/huya.py
@@ -98,9 +98,13 @@ def _get_streams(self):
self.id, self.author, self.title, streamdata = data
for cdntype, priority, streamname, flvurl, suffix, anticode in streamdata:
+ url = update_scheme("https://", f"{flvurl}/{streamname}.{suffix}?{anticode}")
+ if self.session.http.head(url, raise_for_status=False).status_code >= 400:
+ continue
+
name = f"source_{cdntype.lower()}"
self.QUALITY_WEIGHTS[name] = priority
- yield name, HTTPStream(self.session, update_scheme("https://", f"{flvurl}/{streamname}.{suffix}?{anticode}"))
+ yield name, HTTPStream(self.session, url)
log.debug(f"QUALITY_WEIGHTS: {self.QUALITY_WEIGHTS!r}")
| plugins.huya: 403 Client Error: Forbidden for url
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
streamlink 6.5.0
### Description
```
C:\Users\Admin>streamlink --loglevel=debug https://www.huya.com/lovemia best -o X;\13.flv
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.8.18+
[cli][debug] OpenSSL: OpenSSL 1.1.1u 30 May 2023
[cli][debug] Streamlink: 6.5.0
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.11.17
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.3
[cli][debug] pycountry: 23.12.11
[cli][debug] pycryptodome: 3.19.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.23.2
[cli][debug] trio-websocket: 0.11.1
[cli][debug] typing-extensions: 4.9.0
[cli][debug] urllib3: 2.1.0
[cli][debug] websocket-client: 1.7.0
[cli][debug] Arguments:
[cli][debug] url=https://www.huya.com/lovemia
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --output=X;\13.flv
[cli][debug] --ffmpeg-ffmpeg=C:\Program Files\Streamlink\ffmpeg\ffmpeg.exe
[cli][info] Found matching plugin huya for URL https://www.huya.com/lovemia
[plugins.huya][debug] QUALITY_WEIGHTS: {'source_al': 100, 'source_tx': -1, 'source_hw': -1, 'source_hs': 0}
[cli][info] Available streams: source_tx (worst), source_hw, source_hs, source_al (best)
[cli][info] Opening stream: source_al (http)
[cli][info] Writing output to
C:\Users\Admin\X;\13.flv
[cli][debug] Checking file output
File X;\13.flv already exists! Overwrite it? [y/N] y
[cli][debug] Pre-buffering 8192 bytes
[cli][debug] Writing stream to output
[cli][info] Stream ended
[cli][info] Closing currently open stream...
```
```
C:\Users\Admin>streamlink --loglevel=debug https://www.huya.com/719318 best -o X;\513.flv
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.8.18+
[cli][debug] OpenSSL: OpenSSL 1.1.1u 30 May 2023
[cli][debug] Streamlink: 6.5.0
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.11.17
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.3
[cli][debug] pycountry: 23.12.11
[cli][debug] pycryptodome: 3.19.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.23.2
[cli][debug] trio-websocket: 0.11.1
[cli][debug] typing-extensions: 4.9.0
[cli][debug] urllib3: 2.1.0
[cli][debug] websocket-client: 1.7.0
[cli][debug] Arguments:
[cli][debug] url=https://www.huya.com/719318
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --output=X;\513.flv
[cli][debug] --ffmpeg-ffmpeg=C:\Program Files\Streamlink\ffmpeg\ffmpeg.exe
[cli][info] Found matching plugin huya for URL https://www.huya.com/719318
[plugins.huya][debug] QUALITY_WEIGHTS: {'source_al': -1, 'source_tx': -1, 'source_hw': 50, 'source_hs': 50}
[cli][info] Available streams: source_al (worst), source_tx, source_hw, source_hs (best)
[cli][info] Opening stream: source_hs (http)
[cli][info] Writing output to
C:\Users\Admin\X;\513.flv
[cli][debug] Checking file output
[cli][error] Try 1/1: Could not open stream <HTTPStream ['http', 'https://hs.flv.huya.com/src/2240853517-2240853517-9624392570641580032-4481830490-10057-A-0-1.flv?wsSecret=10a92e90f6d8f76dba67d27906870b08&wsTime=658fb155&fm=RFdxOEJjSjNoNkRKdDZUWV8kMF8kMV8kMl8kMw%3D%3D&ctype=huya_live&fs=bgct']> (Could not open stream: Unable to open URL: https://hs.flv.huya.com/src/2240853517-2240853517-9624392570641580032-4481830490-10057-A-0-1.flv?wsSecret=10a92e90f6d8f76dba67d27906870b08&wsTime=658fb155&fm=RFdxOEJjSjNoNkRKdDZUWV8kMF8kMV8kMl8kMw%3D%3D&ctype=huya_live&fs=bgct (403 Client Error: Forbidden for url: https://hs.flv.huya.com/src/2240853517-2240853517-9624392570641580032-4481830490-10057-A-0-1.flv?wsSecret=10a92e90f6d8f76dba67d27906870b08&wsTime=658fb155&fm=RFdxOEJjSjNoNkRKdDZUWV8kMF8kMV8kMl8kMw%3D%3D&ctype=huya_live&fs=bgct))
error: Could not open stream <HTTPStream ['http', 'https://hs.flv.huya.com/src/2240853517-2240853517-9624392570641580032-4481830490-10057-A-0-1.flv?wsSecret=10a92e90f6d8f76dba67d27906870b08&wsTime=658fb155&fm=RFdxOEJjSjNoNkRKdDZUWV8kMF8kMV8kMl8kMw%3D%3D&ctype=huya_live&fs=bgct']>, tried 1 times, exiting
```
### Debug log
```text
C:\Users\Admin>streamlink --loglevel=debug https://www.huya.com/lovemia best -o X;\13.flv
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.8.18+
[cli][debug] OpenSSL: OpenSSL 1.1.1u 30 May 2023
[cli][debug] Streamlink: 6.5.0
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.11.17
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.3
[cli][debug] pycountry: 23.12.11
[cli][debug] pycryptodome: 3.19.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.23.2
[cli][debug] trio-websocket: 0.11.1
[cli][debug] typing-extensions: 4.9.0
[cli][debug] urllib3: 2.1.0
[cli][debug] websocket-client: 1.7.0
[cli][debug] Arguments:
[cli][debug] url=https://www.huya.com/lovemia
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --output=X;\13.flv
[cli][debug] --ffmpeg-ffmpeg=C:\Program Files\Streamlink\ffmpeg\ffmpeg.exe
[cli][info] Found matching plugin huya for URL https://www.huya.com/lovemia
[plugins.huya][debug] QUALITY_WEIGHTS: {'source_al': 100, 'source_tx': -1, 'source_hw': -1, 'source_hs': 0}
[cli][info] Available streams: source_tx (worst), source_hw, source_hs, source_al (best)
[cli][info] Opening stream: source_al (http)
[cli][info] Writing output to
C:\Users\Admin\X;\13.flv
[cli][debug] Checking file output
File X;\13.flv already exists! Overwrite it? [y/N] y
[cli][debug] Pre-buffering 8192 bytes
[cli][debug] Writing stream to output
[cli][info] Stream ended
[cli][info] Closing currently open stream...
C:\Users\Admin>streamlink --loglevel=debug https://www.huya.com/719318 best -o X;\513.flv
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.8.18+
[cli][debug] OpenSSL: OpenSSL 1.1.1u 30 May 2023
[cli][debug] Streamlink: 6.5.0
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.11.17
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.3
[cli][debug] pycountry: 23.12.11
[cli][debug] pycryptodome: 3.19.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.23.2
[cli][debug] trio-websocket: 0.11.1
[cli][debug] typing-extensions: 4.9.0
[cli][debug] urllib3: 2.1.0
[cli][debug] websocket-client: 1.7.0
[cli][debug] Arguments:
[cli][debug] url=https://www.huya.com/719318
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --output=X;\513.flv
[cli][debug] --ffmpeg-ffmpeg=C:\Program Files\Streamlink\ffmpeg\ffmpeg.exe
[cli][info] Found matching plugin huya for URL https://www.huya.com/719318
[plugins.huya][debug] QUALITY_WEIGHTS: {'source_al': -1, 'source_tx': -1, 'source_hw': 50, 'source_hs': 50}
[cli][info] Available streams: source_al (worst), source_tx, source_hw, source_hs (best)
[cli][info] Opening stream: source_hs (http)
[cli][info] Writing output to
C:\Users\Admin\X;\513.flv
[cli][debug] Checking file output
[cli][error] Try 1/1: Could not open stream <HTTPStream ['http', 'https://hs.flv.huya.com/src/2240853517-2240853517-9624392570641580032-4481830490-10057-A-0-1.flv?wsSecret=10a92e90f6d8f76dba67d27906870b08&wsTime=658fb155&fm=RFdxOEJjSjNoNkRKdDZUWV8kMF8kMV8kMl8kMw%3D%3D&ctype=huya_live&fs=bgct']> (Could not open stream: Unable to open URL: https://hs.flv.huya.com/src/2240853517-2240853517-9624392570641580032-4481830490-10057-A-0-1.flv?wsSecret=10a92e90f6d8f76dba67d27906870b08&wsTime=658fb155&fm=RFdxOEJjSjNoNkRKdDZUWV8kMF8kMV8kMl8kMw%3D%3D&ctype=huya_live&fs=bgct (403 Client Error: Forbidden for url: https://hs.flv.huya.com/src/2240853517-2240853517-9624392570641580032-4481830490-10057-A-0-1.flv?wsSecret=10a92e90f6d8f76dba67d27906870b08&wsTime=658fb155&fm=RFdxOEJjSjNoNkRKdDZUWV8kMF8kMV8kMl8kMw%3D%3D&ctype=huya_live&fs=bgct))
error: Could not open stream <HTTPStream ['http', 'https://hs.flv.huya.com/src/2240853517-2240853517-9624392570641580032-4481830490-10057-A-0-1.flv?wsSecret=10a92e90f6d8f76dba67d27906870b08&wsTime=658fb155&fm=RFdxOEJjSjNoNkRKdDZUWV8kMF8kMV8kMl8kMw%3D%3D&ctype=huya_live&fs=bgct']>, tried 1 times, exiting
```
| Not a streamlink issue.
As you can see in the list of returned streams, those streams are not different qualities, but streams served on different CDNs. They are also sorted by the CDN quality weights returned by their API. Apparently, only one CDN seems to be working for some reason and not even the one with the highest quality weight.
`source_hw` was working perfectly fine:
```
$ streamlink 'https://www.huya.com/719318' source_hw --player-no-close
[cli][info] Found matching plugin huya for URL https://www.huya.com/719318
[cli][info] Available streams: source_al (worst), source_tx, source_hw, source_hs (best)
[cli][info] Opening stream: source_hw (http)
[cli][info] Starting player: /usr/bin/mpv
[cli][info] Stream ended
[cli][info] Closing currently open stream...
```
Simple check which sends a `HEAD` HTTP request to each stream URL:
```
$ streamlink \
--json \
'https://www.huya.com/719318' \
| jq -r ".streams | to_entries[] | \"\(.key) '\(.value.url)'\"" \
| xargs -n2 bash -c 'echo "STREAM: $1";curl -I "$2"' --
STREAM: source_al
HTTP/1.1 403 Forbidden
Server: Tengine
Date: Sat, 30 Dec 2023 10:19:43 GMT
Content-Type: text/html
Content-Length: 254
Connection: close
X-Tengine-Type: live
cdncip: redacted
cdnsip: 47.246.48.98
Access-Control-Expose-Headers: alt-svc,cdncip,cdnsip
Access-Control-Allow-Origin: *
Via: cache5.nl2[,0]
STREAM: source_tx
HTTP/1.1 404 Not Found
Server: MC_VCLOUD_LIVE
Date: Sat, 30 Dec 2023 10:19:44 GMT
Content-Length: 0
Connection: keep-alive
Cache-Control: max-age=0
Access-Control-Allow-Credentials: true
Access-Control-Allow-Origin: *
X-Tlive-SpanId: 620A216529CA21CA
Access-Control-Expose-Headers: alt-svc,cdncip,cdnsip
cdncip: redacted
cdnsip: 101.33.10.98
X-NWS-LOG-UUID: 2bc8460d-4500-4618-ae90-05b7ad907d89
X-SSL-PROTOCOL: TLSv1.3
STREAM: source_hw
HTTP/1.1 302 Moved Temporarily
Server: openresty
Date: Sat, 30 Dec 2023 10:19:46 GMT
Connection: close
Access-Control-Expose-Headers: alt-svc, cdncip, cdnsip
cdncip: redacted
cdnsip: 2408:8756:d0ff:40::39
Location: https://4c5f25b40eb0e7fd16f4b6a11caeede4.livehwc3.cn/hw.flv.huya.com/huyalive/2240853517-2240853517-9624392570641580032-4481830490-10057-A-0-1.flv?wsTime=658feebd&cdn_redirect_tag=1703931586&cdn_redirect_domain=ed60e6e67f460afe5345e98fff768af4.livehwc3.cn&policy=6&fm=RFdxOEJjSjNoNkRKdDZUWV8kMF8kMV8kMl8kMw%3D%3D&ctype=huya_live&fs=bgct&cdn_redirect=true&wsSecret=2eb3589f07e256abdc06cd4e3e15b359
Access-Control-Allow-Origin: *
Access-Control-Allow-Methods: GET, POST, OPTIONS
Access-Control-Allow-Headers: DNT,X-Mx-ReqToken,Keep-Alive,User-Agent,X-Requested-With,If-Modified-Since,Cache-Control,Content-Type,Authorization
STREAM: source_hs
HTTP/2 403
access-control-allow-methods: GET,POST,OPTIONS
access-control-allow-origin: *
access-control-expose-headers: alt-svc, cdncip, cdnsip
cache-control: no-cache
content-type: video/x-flv
server: Bytedance NSS
via: n045-043-034-227
x-has-token: 29UWy/Pyqhkn6+SCJdomWFOWv+Z3ek+It3lLy+tPcrfz67n5p8zH5Imd
x-server-ip: 45.43.34.227
cdncip: redacted
cdnsip: 2602:ffe4:2:16::7
date: Sat, 30 Dec 2023 10:19:47 GMT
```
The plugin could do the same and check the HTTP status of each available stream, so non-working ones could be filtered out. | 2023-12-30T11:50:22 |
|
streamlink/streamlink | 5,754 | streamlink__streamlink-5754 | [
"5753"
] | fb953fbc0fc8d09fbed5f1cd24b6543c13e253c4 | diff --git a/src/streamlink/plugins/bigo.py b/src/streamlink/plugins/bigo.py
--- a/src/streamlink/plugins/bigo.py
+++ b/src/streamlink/plugins/bigo.py
@@ -1,41 +1,68 @@
"""
-$description Global live streaming platform for live video game broadcasts and individual live streams.
-$url live.bigo.tv
-$url bigoweb.co
+$description Global live-streaming platform for live video game broadcasts and individual live streams.
+$url bigo.tv
$type live
+$metadata id
+$metadata author
+$metadata category
+$metadata title
"""
+import logging
import re
from streamlink.plugin import Plugin, pluginmatcher
-from streamlink.plugin.api import useragents, validate
+from streamlink.plugin.api import validate
from streamlink.stream.hls import HLSStream
+log = logging.getLogger(__name__)
+
+
@pluginmatcher(re.compile(
- r"https?://(?:www\.)?bigo\.tv/([^/]+)$",
+ r"https?://(?:www\.)?bigo\.tv/(?P<site_id>[^/]+)$",
))
class Bigo(Plugin):
- _api_url = "https://www.bigo.tv/OInterface/getVideoParam?bigoId={0}"
-
- _video_info_schema = validate.Schema({
- "code": 0,
- "msg": "success",
- "data": {
- "videoSrc": validate.any(None, "", validate.url()),
- },
- })
+ _URL_API = "https://ta.bigo.tv/official_website/studio/getInternalStudioInfo"
def _get_streams(self):
- res = self.session.http.get(
- self._api_url.format(self.match.group(1)),
- allow_redirects=True,
- headers={"User-Agent": useragents.IPHONE_6},
+ self.id, self.author, self.category, self.title, hls_url = self.session.http.post(
+ self._URL_API,
+ params={
+ "siteId": self.match["site_id"],
+ "verify": "",
+ },
+ schema=validate.Schema(
+ validate.parse_json(),
+ {
+ "code": 0,
+ "msg": "success",
+ "data": {
+ "roomId": validate.any(None, str),
+ "clientBigoId": validate.any(None, str),
+ "gameTitle": str,
+ "roomTopic": str,
+ "hls_src": validate.any(None, "", validate.url()),
+ },
+ },
+ validate.union_get(
+ ("data", "roomId"),
+ ("data", "clientBigoId"),
+ ("data", "gameTitle"),
+ ("data", "roomTopic"),
+ ("data", "hls_src"),
+ ),
+ ),
)
- data = self.session.http.json(res, schema=self._video_info_schema)
- videourl = data["data"]["videoSrc"]
- if videourl:
- yield "live", HLSStream(self.session, videourl)
+
+ if not self.id:
+ return
+
+ if not hls_url:
+ log.info("Channel is offline")
+ return
+
+ yield "live", HLSStream(self.session, hls_url)
__plugin__ = Bigo
| diff --git a/tests/plugins/test_bigo.py b/tests/plugins/test_bigo.py
--- a/tests/plugins/test_bigo.py
+++ b/tests/plugins/test_bigo.py
@@ -6,27 +6,5 @@ class TestPluginCanHandleUrlBigo(PluginCanHandleUrl):
__plugin__ = Bigo
should_match = [
- "http://bigo.tv/00000000",
- "https://bigo.tv/00000000",
- "https://www.bigo.tv/00000000",
- "http://www.bigo.tv/00000000",
- "http://www.bigo.tv/fancy1234",
- "http://www.bigo.tv/abc.123",
- "http://www.bigo.tv/000000.00",
- ]
-
- should_not_match = [
- # Old URLs don't work anymore
- "http://live.bigo.tv/00000000",
- "https://live.bigo.tv/00000000",
- "http://www.bigoweb.co/show/00000000",
- "https://www.bigoweb.co/show/00000000",
- "http://bigoweb.co/show/00000000",
- "https://bigoweb.co/show/00000000",
-
- # Wrong URL structure
- "https://www.bigo.tv/show/00000000",
- "http://www.bigo.tv/show/00000000",
- "http://bigo.tv/show/00000000",
- "https://bigo.tv/show/00000000",
+ "https://www.bigo.tv/SITE_ID",
]
| plugins.bigo: Unable to parse JSON
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest release
### Description
Hello,
the bigo.py is not working at the moment.
It is giving a parse JSON error.
Debug log is following...
### Debug log
```text
error: Unable to parse JSON: Expecting value: line 1 column 1 (char 0) ('<!DOCTYPE html>\n<html lang="en" s ...)
```
| 2024-01-03T11:26:44 |
|
streamlink/streamlink | 5,757 | streamlink__streamlink-5757 | [
"5756"
] | 1d7fc8d068da6d99c13f9211677c0fccf4f48cb4 | diff --git a/src/streamlink_cli/output/player.py b/src/streamlink_cli/output/player.py
--- a/src/streamlink_cli/output/player.py
+++ b/src/streamlink_cli/output/player.py
@@ -235,8 +235,11 @@ def _open(self):
warnings.warn(
"\n".join([
"The --player argument has been changed and now only takes player path values:",
- " Player paths with spaces don't have to be wrapped in quotation marks anymore.",
- " Custom player arguments need to be set via --player-args.",
+ " Player paths must not be wrapped in additional quotation marks",
+ " and custom player arguments need to be set via --player-args.",
+ " This is most likely caused by using an old config file from an ealier Streamlink version.",
+ " Please see the migration guides in Streamlink's documentation:",
+ " https://streamlink.github.io/migrations.html#player-path-only-player-cli-argument",
]),
StreamlinkWarning,
stacklevel=1,
| Streamlink cannot find player executable on Windows 11
### Checklist
- [X] This is a bug report and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed bug reports](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22bug%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
6.5.0
### Description
On Windows 11, build 22631, I'm trying to use Streamlink with MPC-HC64, but it cannot find the player executable despite being copy pasted into configuration as shown in the image.

This worked some small number of months ago.
### Debug log
```text
PS C:\Users\anthony> streamlink https://www.youtube.com/watch?v=grdawJ3icdA best --loglevel=debug
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.11.7
[cli][debug] OpenSSL: OpenSSL 3.0.11 19 Sep 2023
[cli][debug] Streamlink: 6.5.0
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.11.17
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.3
[cli][debug] pycountry: 23.12.11
[cli][debug] pycryptodome: 3.19.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.23.2
[cli][debug] trio-websocket: 0.11.1
[cli][debug] typing-extensions: 4.9.0
[cli][debug] urllib3: 2.1.0
[cli][debug] websocket-client: 1.7.0
[cli][debug] Arguments:
[cli][debug] url=https://www.youtube.com/watch?v=grdawJ3icdA
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --player="C:\Program Files (x86)\K-Lite Codec Pack\MPC-HC64\mpc-hc64.exe"
[cli][debug] --ffmpeg-ffmpeg=C:\Program Files\Streamlink\ffmpeg\ffmpeg.exe
[cli][info] Found matching plugin youtube for URL https://www.youtube.com/watch?v=grdawJ3icdA
[plugins.youtube][debug] Using video ID: grdawJ3icdA
[stream.ffmpegmux][debug] ffmpeg version n6.1-20231112 Copyright (c) 2000-2023 the FFmpeg developers
[stream.ffmpegmux][debug] built with gcc 13.2.0 (crosstool-NG 1.25.0.232_c175b21)
[stream.ffmpegmux][debug] configuration: --prefix=/ffbuild/prefix --pkg-config-flags=--static --pkg-config=pkg-config --cross-prefix=x86_64-w64-mingw32- --arch=x86_64 --target-os=mingw32 --enable-gpl --enable-version3 --disable-debug --disable-w32threads --enable-pthreads --enable-iconv --enable-libxml2 --enable-zlib --enable-libfreetype --enable-libfribidi --enable-gmp --enable-lzma --enable-fontconfig --enable-libharfbuzz --enable-libvorbis --enable-opencl --disable-libpulse --enable-libvmaf --disable-libxcb --disable-xlib --enable-amf --enable-libaom --enable-libaribb24 --enable-avisynth --enable-chromaprint --enable-libdav1d --enable-libdavs2 --disable-libfdk-aac --enable-ffnvcodec --enable-cuda-llvm --enable-frei0r --enable-libgme --enable-libkvazaar --enable-libaribcaption --enable-libass --enable-libbluray --enable-libjxl --enable-libmp3lame --enable-libopus --enable-librist --enable-libssh --enable-libtheora --enable-libvpx --enable-libwebp --enable-lv2 --enable-libvpl --enable-openal --enable-libopencore-amrnb --enable-libopencore-amrwb --enable-libopenh264 --enable-libopenjpeg --enable-libopenmpt --enable-librav1e --enable-librubberband --enable-schannel --enable-sdl2 --enable-libsoxr --enable-libsrt --enable-libsvtav1 --enable-libtwolame --enable-libuavs3d --disable-libdrm --enable-vaapi --enable-libvidstab --enable-vulkan --enable-libshaderc --enable-libplacebo --enable-libx264 --enable-libx265 --enable-libxavs2 --enable-libxvid --enable-libzimg --enable-libzvbi --extra-cflags=-DLIBTWOLAME_STATIC --extra-cxxflags= --extra-ldflags=-pthread --extra-ldexeflags= --extra-libs=-lgomp --extra-version=20231112
[stream.ffmpegmux][debug] libavutil 58. 29.100 / 58. 29.100
[stream.ffmpegmux][debug] libavcodec 60. 31.102 / 60. 31.102
[stream.ffmpegmux][debug] libavformat 60. 16.100 / 60. 16.100
[stream.ffmpegmux][debug] libavdevice 60. 3.100 / 60. 3.100
[stream.ffmpegmux][debug] libavfilter 9. 12.100 / 9. 12.100
[stream.ffmpegmux][debug] libswscale 7. 5.100 / 7. 5.100
[stream.ffmpegmux][debug] libswresample 4. 12.100 / 4. 12.100
[stream.ffmpegmux][debug] libpostproc 57. 3.100 / 57. 3.100
[plugins.youtube][debug] MuxedStream: v 137 a 251 = 1080p
[plugins.youtube][debug] MuxedStream: v 135 a 251 = 480p
[plugins.youtube][debug] MuxedStream: v 133 a 251 = 240p
[plugins.youtube][debug] MuxedStream: v 160 a 251 = 144p
[cli][info] Available streams: audio_mp4a, audio_opus, 144p (worst), 240p, 360p, 480p, 720p, 1080p (best)
[cli][info] Opening stream: 1080p (muxed-stream)
[cli][info] Starting player: "C:\Program Files (x86)\K-Lite Codec Pack\MPC-HC64\mpc-hc64.exe"
[stream.ffmpegmux][debug] Opening http substream
[stream.ffmpegmux][debug] Opening http substream
[utils.named_pipe][info] Creating pipe streamlinkpipe-25744-1-7320
[utils.named_pipe][info] Creating pipe streamlinkpipe-25744-2-2945
[stream.ffmpegmux][debug] ffmpeg command: C:\Program Files\Streamlink\ffmpeg\ffmpeg.exe -nostats -y -i \\.\pipe\streamlinkpipe-25744-1-7320 -i \\.\pipe\streamlinkpipe-25744-2-2945 -c:v copy -c:a copy -map 0 -map 1 -f matroska pipe:1
[stream.ffmpegmux][debug] Starting copy to pipe: \\.\pipe\streamlinkpipe-25744-1-7320
[stream.ffmpegmux][debug] Starting copy to pipe: \\.\pipe\streamlinkpipe-25744-2-2945
[cli][debug] Pre-buffering 8192 bytes
[warnings][streamlinkwarning] The --player argument has been changed and now only takes player path values:
Player paths with spaces don't have to be wrapped in quotation marks anymore.
Custom player arguments need to be set via --player-args.
error: Failed to start player: "C:\Program Files (x86)\K-Lite Codec Pack\MPC-HC64\mpc-hc64.exe" (Player executable not found)
[cli][info] Closing currently open stream...
[stream.ffmpegmux][debug] Closing ffmpeg thread
[stream.ffmpegmux][debug] Pipe copy complete: \\.\pipe\streamlinkpipe-25744-1-7320
[stream.ffmpegmux][debug] Pipe copy complete: \\.\pipe\streamlinkpipe-25744-2-2945
[stream.ffmpegmux][debug] Closed all the substreams
PS C:\Users\anthony>
```
| It looks like quotes are not optional now (even if not by explicit design); using a path without quotes seems to work for me, but with quotes does not.
Does without quotes work for you?
Hah, you nailed it right on the head.
Yep, that fixed it. I removed the quotes from my config file and it launched correctly.
Thanks!
More recent versions of Streamlink for Windows install an initial default config that does not include quoted paths in the commented-out example `player` options.
I will reopen this, as the warning message leaves open the possibility that quotes may still be used even if unnecessary, therefore, either the message should be changed or the failure when quotes are used should be investigated and possibly fixed.
> it cannot find the player executable despite being copy pasted into configuration as shown in the image.
Please read the 6.0.0 changelog and the migration guide:
- https://streamlink.github.io/changelog.html#streamlink-6-0-0-2023-07-20
- https://streamlink.github.io/migrations.html#streamlink-6-0-0
Streamlink's Windows installer never updates the user's config file. You however have the option to overwrite it with a new config file.
https://github.com/streamlink/windows-builds#notes
> or the failure when quotes are used should be investigated and possibly fixed
This can't be fixed, as explained in https://github.com/streamlink/streamlink/issues/5305#issuecomment-1527696916
We can change the warning message though: `s/don't have to/must not/` | 2024-01-03T22:59:19 |
|
streamlink/streamlink | 5,758 | streamlink__streamlink-5758 | [
"5600"
] | 1d7fc8d068da6d99c13f9211677c0fccf4f48cb4 | diff --git a/src/streamlink/plugins/twitch.py b/src/streamlink/plugins/twitch.py
--- a/src/streamlink/plugins/twitch.py
+++ b/src/streamlink/plugins/twitch.py
@@ -565,7 +565,12 @@ async def acquire_client_integrity_token(client: CDPClient):
return await client_session.evaluate(js_get_integrity_token, timeout=eval_timeout)
try:
- client_integrity: Optional[str] = CDPClient.launch(session, acquire_client_integrity_token)
+ client_integrity: Optional[str] = CDPClient.launch(
+ session,
+ acquire_client_integrity_token,
+ # headless mode gets detected by Twitch, so we have to disable it regardless the user config
+ headless=False,
+ )
except WebbrowserError as err:
log.error(f"{type(err).__name__}: {err}")
return None
| plugins.twitch: is_bad_bot=true in headless mode (forced integrity-token acquirement)
### Checklist
- [X] This is a bug report and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed bug reports](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22bug%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
in the latest version client integrity token fetching is disabled, this is a specific version.
### Description
i have a specific version of streamlink that tries to fetch integrity tokens regardless. same exact commands used to work, now suddenly i am getting bad integrity tokens.
i am guessing they might have changed something in their protocol so that the current solution (https://github.com/streamlink/streamlink/issues/5380) might not work when enabled.
### Debug log
```text
streamlink --loglevel=debug https://www.twitch.tv/thebausffs best
[cli][debug] OS: macOS 12.4
[cli][debug] Python: 3.10.5
[cli][debug] OpenSSL: OpenSSL 1.1.1n 15 Mar 2022
[cli][debug] Streamlink: 0.0.0+unknown
[cli][debug] Dependencies:
[cli][debug] certifi: 2022.9.14
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.2
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.17
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.28.1
[cli][debug] trio: 0.22.0
[cli][debug] trio-websocket: 0.10.2
[cli][debug] typing-extensions: 4.3.0
[cli][debug] urllib3: 1.26.12
[cli][debug] websocket-client: 1.4.1
[cli][debug] Arguments:
[cli][debug] url=https://www.twitch.tv/thebausffs
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][info] Found matching plugin twitch for URL https://www.twitch.tv/thebausffs
[plugins.twitch][debug] Getting live HLS streams for thebausffs
[plugins.twitch][info] Acquiring new client-integrity token...
[webbrowser.webbrowser][info] Launching web browser: /Applications/Google Chrome.app/Contents/MacOS/Google Chrome
[webbrowser.webbrowser][debug] Waiting for web browser process to terminate
[plugins.twitch][debug] Client-Integrity token: {"client_id":"<redacted>","client_ip":"<redacted>","device_id":"<redacted>","exp":"2023-10-10T00:56:42Z","iat":"2023-10-09T08:56:42Z","is_bad_bot":"true","iss":"Twitch Client Integrity","nbf":"2023-10-09T08:56:42Z","user_id":""}
[plugins.twitch][info] Is bad bot? True
[plugins.twitch][warning] No client-integrity token acquired
[plugins.twitch][debug] {'adblock': False, 'geoblock_reason': '', 'hide_ads': False, 'server_ads': True, 'show_ads': True}
[utils.l10n][debug] Language code: en_US
error: No playable streams found on this URL: https://www.twitch.tv/thebausffs
```
| Kasada has seemingly started detecting Chrome's new headless mode, it was previously undetected. Visiting Twitch directly in headless mode also results in a bad bot token, so it doesn't have anything to do with streamlink's implementation.
Acquiring a token still works with `--webbrowser-headless false`.
alright, did not know i had headless true by default. yeah only headless seems to be detected π following worked:
```
streamlink --loglevel=debug --webbrowser-headless false https://www.twitch.tv/thebausffs best
```
We could change the default value to `False`, but I'm not entirely sure if that's a good idea.
I had a generic `webbrowser://URL` plugin in the works after implementing the webbrowser API in 6.0.0, but that still hasn't been finished, and TBH, I totally forgot about it. As you know, the Twitch plugin attempts to open streams without an integrity-token first. If we'd set the default value of `--webbrowser-headless` to `False`, then this would mean that for regular webbrowser API usages where the website doesn't detect headless mode, this would be an inferior experience unless the user sets the option to `True` manually. On the other hand, the Twitch plugin is (so far) the only reason why the webbrowser API was added, and if Twitch detects headless mode, having the default value set to `True` is useless, because the user needs to change config option to actually make it work.
So the question is, does setting the default value to `False` make sense for the sake of compatibility, or would it be bad because of an inferior user experience (in other future plugin implementations)?
----
> [cli][debug] Streamlink: 0.0.0+unknown
This version string does only get shown if you messed up your Streamlink install. You either need to clone the git repo and fetch at least one tag while having Streamlink installed in editable mode, or you need to install from the `sdist` tarball, as the correct version is built in there, same as the `bdist` wheels. Don't use the tarball created by GitHub from the tag's snapshot, as it's lacking the necessary info.
And in order to force the integrity-token, you don't need a "custom version of Streamlink" at all. All you need is a simple plugin override while [sideloading](https://streamlink.github.io/cli/plugin-sideloading.html) it.
```diff
diff --git a/src/streamlink/plugins/twitch.py b/src/streamlink/plugins/twitch.py
index e74e901a..d9efb307 100644
--- a/src/streamlink/plugins/twitch.py
+++ b/src/streamlink/plugins/twitch.py
@@ -787,7 +787,7 @@ class Twitch(Plugin):
response, *data = self.api.access_token(is_live, channel_or_vod)
# try again with a client-integrity token if the API response was erroneous
- if response != "token":
+ if True or response != "token":
client_integrity = self._client_integrity_token(channel_or_vod) if is_live else None
response, *data = self.api.access_token(is_live, channel_or_vod, client_integrity)
``` | 2024-01-04T14:05:50 |
|
streamlink/streamlink | 5,762 | streamlink__streamlink-5762 | [
"5761"
] | 27087e0b6d61de4507328e2d29a1801dfb67fa78 | diff --git a/src/streamlink/plugins/vidio.py b/src/streamlink/plugins/vidio.py
--- a/src/streamlink/plugins/vidio.py
+++ b/src/streamlink/plugins/vidio.py
@@ -6,6 +6,7 @@
import logging
import re
from urllib.parse import urlsplit, urlunsplit
+from uuid import uuid4
from streamlink.plugin import Plugin, pluginmatcher
from streamlink.plugin.api import validate
@@ -17,7 +18,7 @@
@pluginmatcher(re.compile(
- r"https?://(?:www\.)?vidio\.com/",
+ r"https?://(?:www\.)?vidio\.com/.+",
))
class Vidio(Plugin):
tokens_url = "https://www.vidio.com/live/{id}/tokens"
@@ -28,6 +29,10 @@ def _get_stream_token(self, stream_id, stream_type):
self.tokens_url.format(id=stream_id),
params={"type": stream_type},
headers={"Referer": self.url},
+ cookies={
+ "ahoy_visit": str(uuid4()),
+ "ahoy_visitor": str(uuid4()),
+ },
schema=validate.Schema(
validate.parse_json(),
{"token": str},
| diff --git a/tests/plugins/test_vidio.py b/tests/plugins/test_vidio.py
--- a/tests/plugins/test_vidio.py
+++ b/tests/plugins/test_vidio.py
@@ -12,6 +12,5 @@ class TestPluginCanHandleUrlVidio(PluginCanHandleUrl):
]
should_not_match = [
- "http://www.vidio.com",
- "https://www.vidio.com",
+ "https://www.vidio.com/",
]
| plugins.vidio: 403 Client Error on stream token acquirement
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Unable to open URL: https://www.vidio.com/live/204/tokens (403 Client Error: Forbidden for url: https://www.vidio.com/live/204/tokens?type=hls)
### Description
The live stream: https://www.vidio.com/live/204-sctv
the output: https://www.vidio.com/live/204/tokens (403 Client Error: Forbidden for url: https://www.vidio.com/live/204/tokens?type=hls)
It is missing sctv
### Debug log
```text
streamlink https://www.vidio.com/live/204-sctv best
[cli][info] Found matching plugin vidio for URL https://www.vidio.com/live/204-sctv
error: Unable to open URL: https://www.vidio.com/live/204/tokens (403 Client Error: Forbidden for url: https://www.vidio.com/live/204/tokens?type=hls)
```
| 2024-01-08T03:55:07 |
|
streamlink/streamlink | 5,771 | streamlink__streamlink-5771 | [
"5770"
] | 9cf393e3bb8842b92545d3696c7ff410946798ee | diff --git a/src/streamlink/plugins/bilibili.py b/src/streamlink/plugins/bilibili.py
--- a/src/streamlink/plugins/bilibili.py
+++ b/src/streamlink/plugins/bilibili.py
@@ -4,23 +4,31 @@
$type live
"""
+import logging
import re
+from streamlink.exceptions import NoStreamsError
from streamlink.plugin import Plugin, pluginmatcher
from streamlink.plugin.api import validate
from streamlink.stream.hls import HLSStream
+log = logging.getLogger(__name__)
+
+
@pluginmatcher(re.compile(
r"https?://live\.bilibili\.com/(?P<channel>[^/]+)",
))
class Bilibili(Plugin):
+ _URL_API_PLAYINFO = "https://api.live.bilibili.com/xlive/web-room/v2/index/getRoomPlayInfo"
+
SHOW_STATUS_OFFLINE = 0
SHOW_STATUS_ONLINE = 1
SHOW_STATUS_ROUND = 2
- def _get_streams(self):
- schema_stream = validate.all(
+ @staticmethod
+ def _schema_streams():
+ return validate.all(
[{
"protocol_name": str,
"format": validate.all(
@@ -44,6 +52,38 @@ def _get_streams(self):
validate.filter(lambda item: item["protocol_name"] == "http_hls"),
)
+ def _get_api_playinfo(self, room_id):
+ return self.session.http.get(
+ self._URL_API_PLAYINFO,
+ params={
+ "room_id": room_id,
+ "no_playurl": 0,
+ "mask": 1,
+ "qn": 0,
+ "platform": "web",
+ "protocol": "0,1",
+ "format": "0,1,2",
+ "codec": "0,1,2",
+ "dolby": 5,
+ "panorama": 1,
+ },
+ schema=validate.Schema(
+ validate.parse_json(),
+ {
+ "code": 0,
+ "data": {
+ "playurl_info": {
+ "playurl": {
+ "stream": self._schema_streams(),
+ },
+ },
+ },
+ },
+ validate.get(("data", "playurl_info", "playurl", "stream")),
+ ),
+ )
+
+ def _get_page_playinfo(self):
data = self.session.http.get(
self.url,
schema=validate.Schema(
@@ -58,7 +98,7 @@ def _get_streams(self):
"live_status": int,
"playurl_info": {
"playurl": {
- "stream": schema_stream,
+ "stream": self._schema_streams(),
},
},
},
@@ -94,9 +134,18 @@ def _get_streams(self):
self.id, self.author, self.category, self.title, live_status, streams = data
if live_status != self.SHOW_STATUS_ONLINE:
- return
+ log.info("Channel is offline")
+ raise NoStreamsError
+
+ return streams
+
+ def _get_streams(self):
+ streams = self._get_page_playinfo()
+ if not streams:
+ log.debug("Falling back to _get_api_playinfo()")
+ streams = self._get_api_playinfo(self.match["channel"])
- for stream in streams:
+ for stream in streams or []:
for stream_format in stream["format"]:
for codec in stream_format["codec"]:
for url_info in codec["url_info"]:
| diff --git a/tests/plugins/test_bilibili.py b/tests/plugins/test_bilibili.py
--- a/tests/plugins/test_bilibili.py
+++ b/tests/plugins/test_bilibili.py
@@ -5,6 +5,6 @@
class TestPluginCanHandleUrlBilibili(PluginCanHandleUrl):
__plugin__ = Bilibili
- should_match = [
- "https://live.bilibili.com/123123123",
+ should_match_groups = [
+ ("https://live.bilibili.com/CHANNEL", {"channel": "CHANNEL"}),
]
| plugins.bilibili: Playable streams can't be found on stream pages with custom layout
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
streamlink 6.5.0
### Description
It appears that for Bilibili streams with custom pages, the plugin will simply say there's no playable stream found, even if a stream is actually live. An example of [a stream with a custom page at the time of posting](https://live.bilibili.com/27888667) is given in the debug log (screenshot shown here).

### Debug log
```text
PS C:\Users\Admin> streamlink --loglevel=debug https://live.bilibili.com/27888667 best
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.11.7
[cli][debug] OpenSSL: OpenSSL 3.0.11 19 Sep 2023
[cli][debug] Streamlink: 6.5.0
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.11.17
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.3
[cli][debug] pycountry: 23.12.11
[cli][debug] pycryptodome: 3.19.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.23.2
[cli][debug] trio-websocket: 0.11.1
[cli][debug] typing-extensions: 4.9.0
[cli][debug] urllib3: 2.1.0
[cli][debug] websocket-client: 1.7.0
[cli][debug] Arguments:
[cli][debug] url=https://live.bilibili.com/27888667
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --ffmpeg-ffmpeg=C:\Program Files\Streamlink\ffmpeg\ffmpeg.exe
[cli][info] Found matching plugin bilibili for URL https://live.bilibili.com/27888667
error: No playable streams found on this URL: https://live.bilibili.com/27888667
```
| 2024-01-13T13:18:26 |
|
streamlink/streamlink | 5,774 | streamlink__streamlink-5774 | [
"5773"
] | 89716761adb198a943da521cb7f7ce00c49aec4d | diff --git a/src/streamlink/plugins/artetv.py b/src/streamlink/plugins/artetv.py
--- a/src/streamlink/plugins/artetv.py
+++ b/src/streamlink/plugins/artetv.py
@@ -2,6 +2,7 @@
$description European public service channel promoting culture, including magazine shows, concerts and documentaries.
$url arte.tv
$type live, vod
+$metadata id
$metadata title
"""
@@ -17,38 +18,41 @@
log = logging.getLogger(__name__)
-@pluginmatcher(re.compile(r"""
- https?://(?:\w+\.)?arte\.tv/(?:guide/)?
- (?P<language>[a-z]{2})/
- (?:
- (?:videos/)?(?P<video_id>(?!RC-|videos)[^/]+?)/.+
- |
- (?:direct|live)
- )
-""", re.VERBOSE))
+@pluginmatcher(
+ name="live",
+ pattern=re.compile(
+ r"https?://(?:\w+\.)?arte\.tv/(?P<language>[a-z]{2})/(?:direct|live)/?",
+ ),
+)
+@pluginmatcher(
+ name="vod",
+ pattern=re.compile(
+ r"https?://(?:\w+\.)?arte\.tv/(?:guide/)?(?P<language>[a-z]{2})/(?:videos/)?(?P<video_id>(?!RC-|videos)[^/]+?)/.+",
+ ),
+)
class ArteTV(Plugin):
- API_URL = "https://api.arte.tv/api/player/v2/config/{0}/{1}"
- API_TOKEN = "MzYyZDYyYmM1Y2Q3ZWRlZWFjMmIyZjZjNTRiMGY4MzY4NzBhOWQ5YjE4MGQ1NGFiODJmOTFlZDQwN2FkOTZjMQ"
+ API_URL = "https://api.arte.tv/api/player/v2/config/{language}/{id}"
def _get_streams(self):
- language = self.match.group("language")
- video_id = self.match.group("video_id")
+ self.id = self.match["video_id"] if self.matches["vod"] else "LIVE"
- json_url = self.API_URL.format(language, video_id or "LIVE")
- headers = {
- "Authorization": f"Bearer {self.API_TOKEN}",
- }
- streams, metadata = self.session.http.get(json_url, headers=headers, schema=validate.Schema(
+ json_url = self.API_URL.format(
+ language=self.match["language"],
+ id=self.id,
+ )
+ streams, metadata = self.session.http.get(json_url, schema=validate.Schema(
validate.parse_json(),
- {"data": {"attributes": {
+ {"data": {"attributes": dict}},
+ validate.get(("data", "attributes")),
+ {
"streams": validate.any(
[],
[
validate.all(
{
- "url": validate.url(),
"slot": int,
- "protocol": validate.any("HLS", "HLS_NG"),
+ "protocol": str,
+ "url": validate.url(),
},
validate.union_get("slot", "protocol", "url"),
),
@@ -58,17 +62,15 @@ def _get_streams(self):
"title": str,
"subtitle": validate.any(None, str),
},
- }}},
- validate.get(("data", "attributes")),
+ },
validate.union_get("streams", "metadata"),
))
- if not streams:
- return
-
self.title = f"{metadata['title']} - {metadata['subtitle']}" if metadata["subtitle"] else metadata["title"]
- for _slot, _protocol, url in sorted(streams, key=itemgetter(0)):
+ for _slot, protocol, url in sorted(streams, key=itemgetter(0)):
+ if "HLS" not in protocol:
+ continue
return HLSStream.parse_variant_playlist(self.session, url)
| diff --git a/tests/plugins/test_artetv.py b/tests/plugins/test_artetv.py
--- a/tests/plugins/test_artetv.py
+++ b/tests/plugins/test_artetv.py
@@ -5,30 +5,51 @@
class TestPluginCanHandleUrlArteTV(PluginCanHandleUrl):
__plugin__ = ArteTV
- should_match = [
- # new url
- "http://www.arte.tv/fr/direct/",
- "http://www.arte.tv/de/live/",
- "http://www.arte.tv/de/videos/074633-001-A/gesprach-mit-raoul-peck",
- "http://www.arte.tv/en/videos/071437-010-A/sunday-soldiers",
- "http://www.arte.tv/fr/videos/074633-001-A/entretien-avec-raoul-peck",
- "http://www.arte.tv/pl/videos/069873-000-A/supermama-i-businesswoman",
+ should_match_groups = [
+ # live
+ (
+ ("live", "https://www.arte.tv/fr/direct"),
+ {"language": "fr"},
+ ),
+ (
+ ("live", "https://www.arte.tv/fr/direct/"),
+ {"language": "fr"},
+ ),
+ (
+ ("live", "https://www.arte.tv/de/live"),
+ {"language": "de"},
+ ),
+ (
+ ("live", "https://www.arte.tv/de/live/"),
+ {"language": "de"},
+ ),
- # old url - some of them get redirected and some are 404
- "http://www.arte.tv/guide/fr/direct",
- "http://www.arte.tv/guide/de/live",
- "http://www.arte.tv/guide/fr/024031-000-A/le-testament-du-docteur-mabuse",
- "http://www.arte.tv/guide/de/024031-000-A/das-testament-des-dr-mabuse",
- "http://www.arte.tv/guide/en/072544-002-A/christmas-carols-from-cork",
- "http://www.arte.tv/guide/es/068380-000-A/una-noche-en-florencia",
- "http://www.arte.tv/guide/pl/068916-006-A/belle-and-sebastian-route-du-rock",
+ # vod
+ (
+ ("vod", "https://www.arte.tv/de/videos/097372-001-A/mysterium-satoshi-bitcoin-wie-alles-begann-1-6/"),
+ {"language": "de", "video_id": "097372-001-A"},
+ ),
+ (
+ ("vod", "https://www.arte.tv/en/videos/097372-001-A/the-satoshi-mystery-the-story-of-bitcoin/"),
+ {"language": "en", "video_id": "097372-001-A"},
+ ),
+
+ # old vod URLs with redirects
+ (
+ ("vod", "https://www.arte.tv/guide/de/097372-001-A/mysterium-satoshi-bitcoin-wie-alles-begann-1-6/"),
+ {"language": "de", "video_id": "097372-001-A"},
+ ),
+ (
+ ("vod", "https://www.arte.tv/guide/en/097372-001-A/the-satoshi-mystery-the-story-of-bitcoin/"),
+ {"language": "en", "video_id": "097372-001-A"},
+ ),
]
should_not_match = [
- # shouldn't match
- "http://www.arte.tv/guide/fr/plus7/",
- "http://www.arte.tv/guide/de/plus7/",
+ "https://www.arte.tv/guide/de/live/",
+ "https://www.arte.tv/guide/fr/plus7/",
+ "https://www.arte.tv/guide/de/plus7/",
# shouldn't match - playlists without video ids in url
- "http://www.arte.tv/en/videos/RC-014457/the-power-of-forests/",
- "http://www.arte.tv/en/videos/RC-013118/street-art/",
+ "https://www.arte.tv/en/videos/RC-014457/the-power-of-forests/",
+ "https://www.arte.tv/en/videos/RC-013118/street-art/",
]
| plugins.artetv: error: Unable to validate response text: ValidationError(dict):
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
streamlink 6.5.0
### Description
I fix this issue
```
by adding '**API_HLS_NG**' in line 51 of file
`/usr/lib/python3.11/site-packages/streamlink/plugins/artetv.py`
like this :
```

link/streamlink/assets/19744191/b78f47ba-67b2-439b-b336-85bef7e4615a)
### Debug log
```text
error: Unable to validate response text: ValidationError(dict):
Unable to validate value of key 'data'
Context(dict):
Unable to validate value of key 'attributes'
Context(dict):
Unable to validate value of key 'streams'
Context(AnySchema):
ValidationError(AnySchema):
ValidationError(AnySchema):
ValidationError(dict):
Unable to validate value of key 'protocol'
Context(AnySchema):
ValidationError(equality):
'API_HLS_NG' does not equal 'HLS'
ValidationError(equality):
'API_HLS_NG' does not equal 'HLS_NG'
```
| 2024-01-14T19:47:24 |
|
streamlink/streamlink | 5,785 | streamlink__streamlink-5785 | [
"5784"
] | 759c29e4710b184927526462ee041d7d00cc363c | diff --git a/src/streamlink/plugins/huya.py b/src/streamlink/plugins/huya.py
--- a/src/streamlink/plugins/huya.py
+++ b/src/streamlink/plugins/huya.py
@@ -12,22 +12,28 @@
import re
from html import unescape as html_unescape
from typing import Dict
+from urllib.parse import parse_qsl
from streamlink.plugin import Plugin, pluginmatcher
from streamlink.plugin.api import validate
from streamlink.stream.http import HTTPStream
-from streamlink.utils.url import update_scheme
+from streamlink.utils.url import update_qsd, update_scheme
log = logging.getLogger(__name__)
@pluginmatcher(re.compile(
- r"https?://(?:www\.)?huya\.com/(?P<channel>[^/]+)",
+ r"https?://(?:www\.)?huya\.com/(?P<channel>[^/?]+)",
))
class Huya(Plugin):
QUALITY_WEIGHTS: Dict[str, int] = {}
+ _QUALITY_WEIGHTS_OVERRIDE = {
+ "source_hy": -1000, # SSLCertVerificationError
+ }
+ _STREAM_URL_QUERYSTRING_PARAMS = "wsSecret", "wsTime"
+
@classmethod
def stream_weight(cls, key):
weight = cls.QUALITY_WEIGHTS.get(key)
@@ -97,13 +103,18 @@ def _get_streams(self):
self.id, self.author, self.title, streamdata = data
+ self.session.http.headers.update({
+ "Origin": "https://www.huya.com",
+ "Referer": "https://www.huya.com/",
+ })
+
for cdntype, priority, streamname, flvurl, suffix, anticode in streamdata:
- url = update_scheme("https://", f"{flvurl}/{streamname}.{suffix}?{anticode}")
- if self.session.http.head(url, raise_for_status=False).status_code >= 400:
- continue
+ qs = {k: v for k, v in dict(parse_qsl(anticode)).items() if k in self._STREAM_URL_QUERYSTRING_PARAMS}
+ url = update_scheme("https://", f"{flvurl}/{streamname}.{suffix}")
+ url = update_qsd(url, qs)
name = f"source_{cdntype.lower()}"
- self.QUALITY_WEIGHTS[name] = priority
+ self.QUALITY_WEIGHTS[name] = self._QUALITY_WEIGHTS_OVERRIDE.get(name, priority)
yield name, HTTPStream(self.session, url)
log.debug(f"QUALITY_WEIGHTS: {self.QUALITY_WEIGHTS!r}")
| diff --git a/tests/plugins/test_huya.py b/tests/plugins/test_huya.py
--- a/tests/plugins/test_huya.py
+++ b/tests/plugins/test_huya.py
@@ -5,14 +5,10 @@
class TestPluginCanHandleUrlHuya(PluginCanHandleUrl):
__plugin__ = Huya
- should_match = [
- "http://www.huya.com/123123123",
- "http://www.huya.com/name",
- "https://www.huya.com/123123123",
- "https://www.huya.com/name",
+ should_match_groups = [
+ ("https://www.huya.com/CHANNEL", {"channel": "CHANNEL"}),
]
should_not_match = [
- "http://www.huya.com",
"https://www.huya.com",
]
| plugins.huya: 403 Client Error: Forbidden for url
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
streamlink 6.5.1
### Description
please forgive me, my english so bad and it's hard to understand all these rules, im newbie inpython, I donβt know, this has already been asked and I read the answer that this is not a streamlink problem, but maybe you can help me
### Debug log
```text
C:\Users\matebeats>streamlink.exe https://www.huya.com/sbwfz best
[cli][info] Found matching plugin huya for URL https://www.huya.com/sbwfz
[cli][info] Available streams: source_hw (worst, best)
[cli][info] Opening stream: source_hw (http)
[cli][info] Starting player: D:\matebeats\soft\media\VLC\vlc.exe
[cli][error] Try 1/1: Could not open stream <HTTPStream ['http', 'https://hw.flv.huya.com/src/70165683-70165683-301359313786503168-140454822-10057-A-0-1.flv?wsSecret=79ab95ca0c616135da585dba0e2373ef&wsTime=65a9468d&fm=RFdxOEJjSjNoNkRKdDZUWV8kMF8kMV8kMl8kMw%3D%3D&ctype=huya_live&fs=bgct']> (Could not open stream: Unable to open URL: https://hw.flv.huya.com/src/70165683-70165683-301359313786503168-140454822-10057-A-0-1.flv?wsSecret=79ab95ca0c616135da585dba0e2373ef&wsTime=65a9468d&fm=RFdxOEJjSjNoNkRKdDZUWV8kMF8kMV8kMl8kMw%3D%3D&ctype=huya_live&fs=bgct (403 Client Error: Forbidden for url: https://7b232e9a7ece13f936b4e19cb1594132.livehwc3.cn/hw.flv.huya.com/src/70165683-70165683-301359313786503168-140454822-10057-A-0-1.flv?cdn_redirect_domain=14769ccad573a2388a716f2ade587c96.livehwc3.cn&cdn_redirect=true&wsTime=65a9468d&ctype=huya_live&fs=bgct&policy=6&fm=RFdxOEJjSjNoNkRKdDZUWV8kMF8kMV8kMl8kMw%3D%3D&wsSecret=79ab95ca0c616135da585dba0e2373ef&cdn_redirect_tag=1705592487))
error: Could not open stream <HTTPStream ['http', 'https://hw.flv.huya.com/src/70165683-70165683-301359313786503168-140454822-10057-A-0-1.flv?wsSecret=79ab95ca0c616135da585dba0e2373ef&wsTime=65a9468d&fm=RFdxOEJjSjNoNkRKdDZUWV8kMF8kMV8kMl8kMw%3D%3D&ctype=huya_live&fs=bgct']>, tried 1 times, exiting
```
| what should I do and where? im replace huya.py but its not work, then I manually deleted where the - was and inserted it where + the stream started for a second, test_huya.py I didn't find the streamlink in the directory, sorry again for my knowledge
> I manually deleted where the - was and inserted it where +
You were looking at the code **diff** format, which is not intended for you to copy and modify by hand.
Just download the full plugin file from here (the "raw" file link) and sideload it:
https://raw.githubusercontent.com/bastimeyer/streamlink/plugins/huya/5784/src/streamlink/plugins/huya.py
More help here:
- https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#pull-request-feedback
- https://streamlink.github.io/latest/cli/plugin-sideloading.html
If you can, please provide feedback with a full **debug log** (`--loglevel=debug`), so we can see that you are correctly sideloading the new plugin. Please check if any streams on this site are not working, because as I said in the pull request, I am not sure if my changes are working on all live streams. Thank you. | 2024-01-18T18:37:45 |
streamlink/streamlink | 5,792 | streamlink__streamlink-5792 | [
"5791"
] | 6fe26dac768b64e3e4d33b51410bb12524860dca | diff --git a/src/streamlink/plugins/aloula.py b/src/streamlink/plugins/aloula.py
--- a/src/streamlink/plugins/aloula.py
+++ b/src/streamlink/plugins/aloula.py
@@ -19,14 +19,14 @@
log = logging.getLogger(__name__)
-@pluginmatcher(re.compile(r"""
- https?://(?:www\.)?aloula\.sa/(?:\w{2}/)?
- (?:
- live/(?P<live_slug>[^/?&]+)
- |
- episode/(?P<vod_id>\d+)
- )
-""", re.VERBOSE))
+@pluginmatcher(
+ name="live",
+ pattern=re.compile(r"https?://(?:www\.)?aloula\.sa/(?:\w{2}/)?live/(?P<live_slug>[^/?&]+)"),
+)
+@pluginmatcher(
+ name="vod",
+ pattern=re.compile(r"https?://(?:www\.)?aloula\.sa/(?:\w{2}/)?episode/(?P<vod_id>\d+)"),
+)
class Aloula(Plugin):
def get_live(self, live_slug):
live_data = self.session.http.get(
@@ -106,13 +106,15 @@ def get_vod(self, vod_id):
return HLSStream.parse_variant_playlist(self.session, hls_url)
def _get_streams(self):
- live_slug = self.match.group("live_slug")
- vod_id = self.match.group("vod_id")
+ self.session.http.headers.update({
+ "Origin": "https://www.aloula.sa",
+ "Referer": "https://www.aloula.sa/",
+ })
- if live_slug:
- return self.get_live(live_slug)
- elif vod_id:
- return self.get_vod(vod_id)
+ if self.matches["live"]:
+ return self.get_live(self.match["live_slug"])
+ else:
+ return self.get_vod(self.match["vod_id"])
__plugin__ = Aloula
| diff --git a/tests/plugins/test_aloula.py b/tests/plugins/test_aloula.py
--- a/tests/plugins/test_aloula.py
+++ b/tests/plugins/test_aloula.py
@@ -6,15 +6,10 @@ class TestPluginCanHandleUrlAloula(PluginCanHandleUrl):
__plugin__ = Aloula
should_match_groups = [
- ("https://www.aloula.sa/live/slug", {"live_slug": "slug"}),
- ("https://www.aloula.sa/en/live/slug", {"live_slug": "slug"}),
- ("https://www.aloula.sa/de/live/slug/abc", {"live_slug": "slug"}),
- ("https://www.aloula.sa/episode/123", {"vod_id": "123"}),
- ("https://www.aloula.sa/en/episode/123", {"vod_id": "123"}),
- ("https://www.aloula.sa/episode/123abc/456", {"vod_id": "123"}),
- ("https://www.aloula.sa/de/episode/123abc/456", {"vod_id": "123"}),
- ("https://www.aloula.sa/episode/123?continue=8", {"vod_id": "123"}),
- ("https://www.aloula.sa/xx/episode/123?continue=8", {"vod_id": "123"}),
+ (("live", "https://www.aloula.sa/live/saudiatv"), {"live_slug": "saudiatv"}),
+ (("live", "https://www.aloula.sa/en/live/saudiatv"), {"live_slug": "saudiatv"}),
+ (("vod", "https://www.aloula.sa/episode/6676"), {"vod_id": "6676"}),
+ (("vod", "https://www.aloula.sa/en/episode/6676"), {"vod_id": "6676"}),
]
should_not_match = [
| plugins.aloula: 403 Client Error: Forbidden for url
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
streamlink 6.5.1
### Description
Can't generate stream URL for aloula plugin with forbidden code (403).
Streamlink can play the stream well on latest versions (using external vlc player). but generating --stream-url fails.
Desktop:~$ streamlink https://www.aloula.sa/en/live/sunnatvsa --stream-url
error: Unable to open URL: https://live.kwikmotion.com/ksasunnalive/ksasunna.smil/playlist_dvr.m3u8?hdnts=exp=1705848372~acl=/ksasunnalive/ksasunna.smil/*~hmac=5ea60ce1704942dc93782e233063d9142c19f4980ea6a4bb856331a08bc73c6c (403 Client Error: Forbidden for url: https://live.kwikmotion.com/ksasunnalive/ksasunna.smil/playlist_dvr.m3u8?hdnts=exp=1705848372~acl=/ksasunnalive/ksasunna.smil/*~hmac=5ea60ce1704942dc93782e233063d9142c19f4980ea6a4bb856331a08bc73c6c)
### Debug log
```text
andalus@Desktop:~$ streamlink https://www.aloula.sa/en/live/sunnatvsa --loglevel=debug
[cli][debug] OS: Linux-5.15.90.1-microsoft-standard-WSL2-x86_64-with-glibc2.35
[cli][debug] Python: 3.10.12
[cli][debug] OpenSSL: OpenSSL 3.0.2 15 Mar 2022
[cli][debug] Streamlink: 6.5.1
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.7.22
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.3
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.19.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.22.2
[cli][debug] trio-websocket: 0.10.4
[cli][debug] typing-extensions: 4.8.0
[cli][debug] urllib3: 2.0.4
[cli][debug] websocket-client: 1.6.3
[cli][debug] Arguments:
[cli][debug] url=https://www.aloula.sa/en/live/sunnatvsa
[cli][debug] --loglevel=debug
[cli][info] Found matching plugin aloula for URL https://www.aloula.sa/en/live/sunnatvsa
[utils.l10n][debug] Language code: en_US
Available streams: 360p (worst), 480p, 1080p (best)
```
| 2024-01-21T14:57:26 |
|
streamlink/streamlink | 5,806 | streamlink__streamlink-5806 | [
"5804"
] | c45bf3c27d5ddbcd62666548a119c876f4977033 | diff --git a/src/streamlink/plugins/qq.py b/src/streamlink/plugins/qq.py
deleted file mode 100644
--- a/src/streamlink/plugins/qq.py
+++ /dev/null
@@ -1,81 +0,0 @@
-"""
-$description Chinese live-streaming platform for live video game broadcasts and live sports game related streams.
-$url live.qq.com
-$type live
-$metadata id
-$metadata author
-$metadata category
-$metadata title
-"""
-
-import logging
-import re
-
-from streamlink.plugin import Plugin, pluginmatcher
-from streamlink.plugin.api import validate
-from streamlink.stream.hls import HLSStream
-from streamlink.utils.url import update_scheme
-
-
-log = logging.getLogger(__name__)
-
-
-@pluginmatcher(re.compile(
- r"https?://(m\.)?live\.qq\.com/(?P<room_id>\d+)?",
-))
-class QQ(Plugin):
- _URL_API = "https://live.qq.com/api/h5/room"
-
- def _get_streams(self):
- room_id = self.match.group("room_id")
-
- if not room_id:
- room_id = self.session.http.get(self.url, schema=validate.Schema(
- validate.parse_html(),
- validate.xml_xpath_string(".//*[@data-rid][1]/@data-rid"),
- ))
- if not room_id:
- return
-
- error, data = self.session.http.get(
- self._URL_API,
- params={"room_id": room_id},
- schema=validate.Schema(
- validate.parse_json(),
- validate.any(
- validate.all(
- {
- "error": 0,
- "data": {
- "nickname": str,
- "game_name": str,
- "room_name": str,
- "hls_url": validate.url(path=validate.endswith(".m3u8")),
- },
- },
- ),
- validate.all(
- {
- "error": int,
- "data": str,
- },
- ),
- ),
- validate.union_get("error", "data"),
- ),
- )
- if error != 0:
- log.error(data)
- return
-
- self.id = room_id
- self.author = data["nickname"]
- self.category = data["game_name"]
- self.title = data["room_name"]
-
- hls_url = update_scheme("https://", data["hls_url"], force=True)
-
- return {"live": HLSStream(self.session, hls_url)}
-
-
-__plugin__ = QQ
| diff --git a/tests/plugins/test_qq.py b/tests/plugins/test_qq.py
deleted file mode 100644
--- a/tests/plugins/test_qq.py
+++ /dev/null
@@ -1,12 +0,0 @@
-from streamlink.plugins.qq import QQ
-from tests.plugins import PluginCanHandleUrl
-
-
-class TestPluginCanHandleUrlQQ(PluginCanHandleUrl):
- __plugin__ = QQ
-
- should_match_groups = [
- ("https://live.qq.com/", {}),
- ("https://live.qq.com/123456789", {"room_id": "123456789"}),
- ("https://m.live.qq.com/123456789", {"room_id": "123456789"}),
- ]
| plugins.qq: 403 Client Error: Forbidden for url
### Checklist
- [X] This is a bug report and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed bug reports](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22bug%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
6.5.0
### Description
seems like i am getting 403 Forbidden when trying to use streamlink for qq.com stream. is this a geo-block issue, as in i need to be in China to be able to access the stream or is the plugin outdated somehow?
### Debug log
```text
streamlink --loglevel=debug https://live.qq.com/10111543 best
[cli][debug] OS: macOS 12.4
[cli][debug] Python: 3.10.5
[cli][debug] OpenSSL: OpenSSL 1.1.1n 15 Mar 2022
[cli][debug] Streamlink: 6.5.0
[cli][debug] Dependencies:
[cli][debug] certifi: 2022.9.14
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.2
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.17
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.28.1
[cli][debug] trio: 0.22.0
[cli][debug] trio-websocket: 0.10.2
[cli][debug] typing-extensions: 4.3.0
[cli][debug] urllib3: 1.26.12
[cli][debug] websocket-client: 1.4.1
[cli][debug] Arguments:
[cli][debug] url=https://live.qq.com/10111543
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][info] Found matching plugin qq for URL https://live.qq.com/10111543
[cli][info] Available streams: live (worst, best)
[cli][info] Opening stream: live (hls)
[cli][info] Starting player: /opt/homebrew/Caskroom/vlc/3.0.18/vlc.wrapper.sh
[stream.hls][debug] Reloading playlist
[cli][debug] Pre-buffering 8192 bytes
[stream.hls][error] Unable to open URL: https://hls-broadcast-bd1.qiecdn.com/live/10111543rySdGBcY.m3u8?timestamp=<redacted>&secret=<redacted>&token=<redacted> (403 Client Error: Forbidden for url: https://hls-broadcast-bd1.qiecdn.com/live/10111543rySdGBcY.m3u8?timestamp=<redacted>&secret=<redacted>&token=<redacted>)
[stream.segmented][debug] Closing worker thread
[stream.segmented][debug] Closing writer thread
[cli][error] Try 1/1: Could not open stream <HLSStream ['hls', 'https://hls-broadcast-bd1.qiecdn.com/live/10111543rySdGBcY.m3u8?timestamp=<redacted>&secret=<redacted>&token=<redacted>']> (No data returned from stream)
error: Could not open stream <HLSStream ['hls', 'https://hls-broadcast-bd1.qiecdn.com/live/10111543rySdGBcY.m3u8?timestamp=<redacted>&secret=<redacted>&token=<redacted>']>, tried 1 times, exiting
[cli][info] Closing currently open stream...
```
| i can access the streams fine on a browser, if that matters for whether it is a geo-block or not.
This site now uses a different API endpoint for getting the stream URL, which is a progressive HTTP stream using an FLV container (why is this always the case with Asian streaming sites?).
Those stream URLs however include a signature parameter which is calculated somewhere deep in their minified/obfuscated JS code, and from what it looks like according to my debugging so far, web assembly is involved here too.
These are the relevant code parts:
- https://static.qiecdn.com/static-next/_next/static/chunks/313.b7190cb8f095d56ab52b.js (entry point is the `m(n, t)` function with `n` being the channel ID and `t` some other integer)
- https://static.qiecdn.com/static-next/_next/static/wasm/1ef6d28bf63c8ccf0cf8.wasm (the `var u=r("oZrZ")` reference)
I don't think it's worth trying to fix this plugin. This is way too much effort to debug this, understand the code, rewrite it in python and hope that they don't make any changes. The only viable alternative I see is implementing Streamlink's webbrowser plugin and trying to intercept the stream URL from the browser's network requests, but this plugin is not worth the effort considering the number of actual users. | 2024-01-30T14:51:48 |
streamlink/streamlink | 5,827 | streamlink__streamlink-5827 | [
"5826"
] | 6cb3c023ab207d37a72f9a03911b632e8ed3fb3b | diff --git a/src/streamlink/plugins/foxtr.py b/src/streamlink/plugins/foxtr.py
deleted file mode 100644
--- a/src/streamlink/plugins/foxtr.py
+++ /dev/null
@@ -1,27 +0,0 @@
-"""
-$description Turkish live TV channel owned by Fox Network.
-$url fox.com.tr
-$type live
-"""
-
-import re
-
-from streamlink.plugin import Plugin, pluginmatcher
-from streamlink.plugin.api import validate
-from streamlink.stream.hls import HLSStream
-
-
-@pluginmatcher(re.compile(
- r"https?://(?:www\.)?fox(?:play)?\.com\.tr/",
-))
-class FoxTR(Plugin):
- def _get_streams(self):
- re_streams = re.compile(r"""(['"])(?P<url>https://\S+/foxtv\.m3u8\S+)\1""")
- res = self.session.http.get(self.url, schema=validate.Schema(
- validate.transform(re_streams.findall),
- ))
- for _, stream_url in res:
- return HLSStream.parse_variant_playlist(self.session, stream_url)
-
-
-__plugin__ = FoxTR
diff --git a/src/streamlink/plugins/nowtvtr.py b/src/streamlink/plugins/nowtvtr.py
new file mode 100644
--- /dev/null
+++ b/src/streamlink/plugins/nowtvtr.py
@@ -0,0 +1,27 @@
+"""
+$description Turkish live TV channel owned by Disney.
+$url nowtv.com.tr
+$type live, vod
+"""
+
+import re
+
+from streamlink.plugin import Plugin, pluginmatcher
+from streamlink.plugin.api import validate
+from streamlink.stream.hls import HLSStream
+
+
+@pluginmatcher(re.compile(
+ r"https?://(?:www\.)?nowtv\.com\.tr/",
+))
+class NowTVTR(Plugin):
+ def _get_streams(self):
+ stream_url = self.session.http.get(self.url, schema=validate.Schema(
+ re.compile(r"""(?P<q>['"])(?P<url>https://nowtv[^/]+/\S+/playlist\.m3u8\?\S+)(?P=q)"""),
+ validate.none_or_all(validate.get("url")),
+ ))
+ if stream_url:
+ return HLSStream.parse_variant_playlist(self.session, stream_url)
+
+
+__plugin__ = NowTVTR
| diff --git a/tests/plugins/test_foxtr.py b/tests/plugins/test_foxtr.py
deleted file mode 100644
--- a/tests/plugins/test_foxtr.py
+++ /dev/null
@@ -1,10 +0,0 @@
-from streamlink.plugins.foxtr import FoxTR
-from tests.plugins import PluginCanHandleUrl
-
-
-class TestPluginCanHandleUrlFoxTR(PluginCanHandleUrl):
- __plugin__ = FoxTR
-
- should_match = [
- "http://www.fox.com.tr/canli-yayin",
- ]
diff --git a/tests/plugins/test_nowtvtr.py b/tests/plugins/test_nowtvtr.py
new file mode 100644
--- /dev/null
+++ b/tests/plugins/test_nowtvtr.py
@@ -0,0 +1,14 @@
+from streamlink.plugins.nowtvtr import NowTVTR
+from tests.plugins import PluginCanHandleUrl
+
+
+class TestPluginCanHandleUrlFoxTR(PluginCanHandleUrl):
+ __plugin__ = NowTVTR
+
+ should_match = [
+ "https://www.nowtv.com.tr/canli-yayin",
+ "https://www.nowtv.com.tr/now-haber",
+ "https://www.nowtv.com.tr/yayin-akisi",
+ "https://www.nowtv.com.tr/Gaddar/bolumler",
+ "https://www.nowtv.com.tr/Memet-Ozer-ile-Mutfakta/bolumler",
+ ]
| plugins.foxtr: name changed to nowtvtr
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
6.5.1
### Description
Hello Team,
As my first message, guidlines and conducts read before, apologies if confusions and thank you for Streamlink tool project.
Since today Turkish Fox channel becomes Now Tv. Website url https://www.fox.com.tr/ name has officially changed to https://www.nowtv.com.tr/
Impacted plugin file location : https://github.com/streamlink/streamlink/blob/master/src/streamlink/plugins/foxtr.py
Could someone update that python file content if possible ? Feel free to feedback me if any please, Thanks a lot, Regards
### Debug log
```text
Debug log precision get on fox.com.tr :
An error occurred: Unable to open URL: https://www.fox.com.tr/canli-yayin?m3u8
(HTTPSConnectionPool(host='www.fox.com.tr', port=443): Max retries exceeded with url: /canli-yayin?m3u8
(Caused by SSLError(SSLCertVerificationError(1, '[SSL: CERTIFICATE_VERIFY_FAILED]
certificate verify failed: unable to get local issuer certificate (_ssl.c:1006)'))))
```
| 2024-02-13T05:17:42 |
|
streamlink/streamlink | 5,844 | streamlink__streamlink-5844 | [
"5843"
] | 61cec0e88e1a49ee09c39b78fa0396c1da3239ea | diff --git a/src/streamlink/session/plugins.py b/src/streamlink/session/plugins.py
--- a/src/streamlink/session/plugins.py
+++ b/src/streamlink/session/plugins.py
@@ -179,7 +179,7 @@ def _load_plugins_from_path(self, path: Union[Path, str]) -> Dict[str, Type[Plug
if lookup is None:
continue
mod, plugin = lookup
- if name in self._plugins:
+ if name in self._plugins or name in self._matchers:
log.info(f"Plugin {name} is being overridden by {mod.__file__}")
plugins[name] = plugin
| diff --git a/tests/session/test_plugins.py b/tests/session/test_plugins.py
--- a/tests/session/test_plugins.py
+++ b/tests/session/test_plugins.py
@@ -131,6 +131,11 @@ def test_load_path_testplugins(self, caplog: pytest.LogCaptureFixture, session:
assert session.plugins["testplugin"].__module__ == "streamlink.plugins.testplugin"
assert caplog.record_tuples == []
+ def test_load_path_testplugins_override(self, caplog: pytest.LogCaptureFixture, session: Streamlink):
+ assert session.plugins.load_path(PATH_TESTPLUGINS)
+ assert "testplugin" in session.plugins
+ assert caplog.record_tuples == []
+
assert session.plugins.load_path(PATH_TESTPLUGINS_OVERRIDE)
assert "testplugin" in session.plugins
assert session.plugins.get_names() == ["testplugin"]
@@ -144,6 +149,26 @@ def test_load_path_testplugins(self, caplog: pytest.LogCaptureFixture, session:
),
]
+ def test_load_path_testplugins_override_matchers(self, caplog: pytest.LogCaptureFixture, session: Streamlink):
+ assert _TestPlugin.matchers
+ session.plugins._matchers.update({"testplugin": _TestPlugin.matchers})
+
+ assert "testplugin" not in session.plugins
+ assert session.plugins.get_names() == ["testplugin"]
+ assert caplog.record_tuples == []
+
+ assert session.plugins.load_path(PATH_TESTPLUGINS)
+ assert session.plugins.get_names() == ["testplugin"]
+ assert session.plugins["testplugin"].__name__ == "TestPlugin"
+ assert session.plugins["testplugin"].__module__ == "streamlink.plugins.testplugin"
+ assert [(record.name, record.levelname, record.message) for record in caplog.records] == [
+ (
+ "streamlink.session",
+ "info",
+ f"Plugin testplugin is being overridden by {PATH_TESTPLUGINS / 'testplugin.py'}",
+ ),
+ ]
+
def test_importerror(self, monkeypatch: pytest.MonkeyPatch, caplog: pytest.LogCaptureFixture, session: Streamlink):
monkeypatch.setattr("importlib.machinery.FileFinder.find_spec", Mock(return_value=None))
assert not session.plugins.load_path(PATH_TESTPLUGINS)
| session.plugins: No longer logs when plugin is overridden
### Checklist
- [X] This is a bug report and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed bug reports](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22bug%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
6.6.1+2.g61cec0e8
### Description
Streamlink no longer logs anything when a plugin is overridden/sideloaded.
It used to log `[session][debug] Plugin twitch is being overridden by ...`
The code seemingly [still exists](https://github.com/streamlink/streamlink/blob/61cec0e88e1a49ee09c39b78fa0396c1da3239ea/src/streamlink/session/plugins.py#L183), but it doesn't get called.
You can see the sideloaded plugin was properly loaded by the log message, but there was no notice by streamlink:
```shell
[hakkin@hakkin-pc ~]$ ls ~/.local/share/streamlink/plugins/
__pycache__ twitch.py
[hakkin@hakkin-pc ~]$ streamlink -o=/dev/null --loglevel=debug --logfile=log.txt https://www.twitch.tv/twitchmedia21 best
...
[plugins.twitch][info] This log is from the overridden plugin!
...
```
### Debug log
```text
[cli][debug] OS: Linux-6.6.16-1-lts-x86_64-with-glibc2.39
[cli][debug] Python: 3.11.7
[cli][debug] OpenSSL: OpenSSL 3.2.1 30 Jan 2024
[cli][debug] Streamlink: 6.6.1+2.g61cec0e8
[cli][debug] Dependencies:
[cli][debug] certifi: 2024.2.2
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 5.1.0
[cli][debug] pycountry: 23.12.11
[cli][debug] pycryptodome: 3.20.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.24.0
[cli][debug] trio-websocket: 0.11.1
[cli][debug] typing-extensions: 4.9.0
[cli][debug] urllib3: 2.2.1
[cli][debug] websocket-client: 1.7.0
[cli][debug] Arguments:
[cli][debug] url=https://www.twitch.tv/twitchmedia21
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --logfile=log.txt
[cli][debug] --output=/dev/null
[plugins.twitch][info] This log is from the overridden plugin!
[cli][info] Found matching plugin twitch for URL https://www.twitch.tv/twitchmedia21
[plugins.twitch][debug] Getting live HLS streams for twitchmedia21
[plugins.twitch][debug] {'adblock': False, 'geoblock_reason': '', 'hide_ads': False, 'server_ads': True, 'show_ads': True}
[utils.l10n][debug] Language code: en_US
[cli][info] Available streams: audio_only, 160p (worst), 360p, 480p, 720p, 720p60, 1080p60 (best)
[cli][info] Opening stream: 1080p60 (hls)
[cli][info] Writing output to
/dev/null
[cli][debug] Checking file output
[stream.hls][debug] Reloading playlist
[cli][debug] Pre-buffering 8192 bytes
[stream.hls][debug] First Sequence: 0; Last Sequence: 3
[stream.hls][debug] Start offset: 0; Duration: None; Start Sequence: 1; End Sequence: None
[stream.hls][debug] Adding segment 1 to queue
[stream.hls][debug] Adding segment 2 to queue
[stream.hls][debug] Adding segment 3 to queue
[stream.hls][debug] Writing segment 1 to output
[stream.hls][debug] Segment 1 complete
[cli][debug] Writing stream to output
[stream.hls][debug] Writing segment 2 to output
[stream.hls][debug] Segment 2 complete
[stream.hls][debug] Writing segment 3 to output
[stream.hls][debug] Segment 3 complete
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Adding segment 4 to queue
[stream.hls][debug] Adding segment 5 to queue
[stream.hls][debug] Adding segment 6 to queue
[stream.hls][debug] Writing segment 4 to output
[stream.hls][debug] Segment 4 complete
[stream.hls][debug] Writing segment 5 to output
[stream.hls][debug] Segment 5 complete
[stream.hls][debug] Writing segment 6 to output
[stream.hls][debug] Segment 6 complete
[stream.segmented][debug] Closing worker thread
[stream.segmented][debug] Closing writer thread
[stream.segmented][debug] Closing writer thread
[cli][info] Stream ended
Interrupted! Exiting...
[cli][info] Closing currently open stream...
```
| 2024-02-20T06:53:45 |
|
streamlink/streamlink | 5,848 | streamlink__streamlink-5848 | [
"5846"
] | 73db061c1df17e9e8fc02cc23a345895e45dfee8 | diff --git a/src/streamlink/plugins/hiplayer.py b/src/streamlink/plugins/hiplayer.py
--- a/src/streamlink/plugins/hiplayer.py
+++ b/src/streamlink/plugins/hiplayer.py
@@ -1,7 +1,6 @@
"""
$description United Arab Emirates CDN hosting live content for various websites in The Middle East.
$url alwasat.ly
-$url media.gov.kw
$type live
$region various
"""
@@ -19,7 +18,6 @@
@pluginmatcher(name="alwasatly", pattern=re.compile(r"https?://(?:www\.)?alwasat\.ly"))
-@pluginmatcher(name="mediagovkw", pattern=re.compile(r"https?://(?:www\.)?media\.gov\.kw"))
class HiPlayer(Plugin):
DAI_URL = "https://pubads.g.doubleclick.net/ssai/event/{0}/streams"
| diff --git a/tests/plugins/test_hiplayer.py b/tests/plugins/test_hiplayer.py
--- a/tests/plugins/test_hiplayer.py
+++ b/tests/plugins/test_hiplayer.py
@@ -7,6 +7,4 @@ class TestPluginCanHandleUrlHiPlayer(PluginCanHandleUrl):
should_match = [
("alwasatly", "https://alwasat.ly/live"),
- ("mediagovkw", "https://www.media.gov.kw/LiveTV.aspx?PanChannel=KTV1"),
- ("mediagovkw", "https://www.media.gov.kw/LiveTV.aspx?PanChannel=KTVSports"),
]
| plugins.hiplayer: new plugin for kuwait tvs changes
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
6.6.1
### Description
Hello,
After few days offlines, change from 'hibridcdn' to 'mangomolo' is noticed for kuwait tv channels.
src/streamlink/plugins/hiplayer.py is no more suitable for : "media.gov.kw" entry
- Still Call page : https://www.media.gov.kw/LiveTV.aspx?PanChannel=KTV1
- Same Channel variants : `KTV1` value could be also : `KTV2, KTVArabe, KhallikBilbait, KTVEthraa, KTVPlus, KTVSports, KTVSportPlus, KTVSportExtra`
Below reported new gathering method details :
- New master src host page : https://player.mangomolo.com/v1/live?id=xxxxxx
- src synthax : https://kwtktv1ta.cdn.mangomolo.com/ktv1/smil:ktv1.stream.smil/playlist.m3u8?xxx
- id ch. variant values : `tv2, tva, kb, eth, ktvaq, sp, spl, alm`
- chunk structure : https://kwtktv1ta.cdn.mangomolo.com/ktv1/smil:ktv1.stream.smil/chunklist_bXXXXX_xyxy==.m3u8
Could someone adapt related plugin please ? Thanks a lot, regards
### Debug log
```text
Currently : Could not find JS URL
```
| https://media.gov.kw is now using a different stream host, namely https://mangomolo.com.
This requires a separate plugin implementation and the hiplayer plugin needs to be updated accordingly with a removal of the unsupported matcher. | 2024-02-20T15:03:24 |
streamlink/streamlink | 5,852 | streamlink__streamlink-5852 | [
"5850"
] | 57dacf7bc9ee1f5793f8aa3c715220ded19653f6 | diff --git a/src/streamlink/plugins/mangomolo.py b/src/streamlink/plugins/mangomolo.py
new file mode 100644
--- /dev/null
+++ b/src/streamlink/plugins/mangomolo.py
@@ -0,0 +1,52 @@
+"""
+$description OTT video platform owned by Alpha Technology Group
+$url media.gov.kw
+$type live
+"""
+
+import logging
+import re
+
+from streamlink.exceptions import NoStreamsError
+from streamlink.plugin import Plugin, pluginmatcher
+from streamlink.plugin.api import validate
+from streamlink.stream import HLSStream
+from streamlink.utils.url import update_scheme
+
+
+log = logging.getLogger(__name__)
+
+
+@pluginmatcher(
+ name="mangomoloplayer",
+ pattern=re.compile(r"https?://player\.mangomolo\.com/v1/"),
+)
+@pluginmatcher(
+ name="mediagovkw",
+ pattern=re.compile(r"https?://media\.gov\.kw/"),
+)
+class Mangomolo(Plugin):
+ def _get_player_url(self):
+ player_url = self.session.http.get(self.url, schema=validate.Schema(
+ validate.parse_html(),
+ validate.xml_xpath_string(".//iframe[contains(@src,'//player.mangomolo.com/v1/')][1]/@src"),
+ ))
+ if not player_url:
+ log.error("Could not find embedded player")
+ raise NoStreamsError
+
+ self.url = update_scheme("https://", player_url)
+
+ def _get_streams(self):
+ if not self.matches["mangomoloplayer"]:
+ self._get_player_url()
+
+ hls_url = self.session.http.get(self.url, schema=validate.Schema(
+ re.compile(r"src\s*:\s*(?P<q>[\"'])(?P<url>https?://\S+?\.m3u8\S*?)(?P=q)"),
+ validate.none_or_all(validate.get("url")),
+ ))
+ if hls_url:
+ return HLSStream.parse_variant_playlist(self.session, hls_url)
+
+
+__plugin__ = Mangomolo
| diff --git a/tests/plugins/test_mangomolo.py b/tests/plugins/test_mangomolo.py
new file mode 100644
--- /dev/null
+++ b/tests/plugins/test_mangomolo.py
@@ -0,0 +1,22 @@
+from streamlink.plugins.mangomolo import Mangomolo
+from tests.plugins import PluginCanHandleUrl
+
+
+class TestPluginCanHandleUrlMangomolo(PluginCanHandleUrl):
+ __plugin__ = Mangomolo
+
+ should_match = [
+ (
+ "mangomoloplayer",
+ "https://player.mangomolo.com/v1/live?id=MTk1&channelid=MzU1&countries=bnVsbA==&w=100%25&h=100%25"
+ + "&autoplay=true&filter=none&signature=9ea6c8ed03b8de6e339d6df2b0685f25&app_id=43",
+ ),
+ (
+ "mediagovkw",
+ "https://media.gov.kw/LiveTV.aspx?PanChannel=KTV1",
+ ),
+ (
+ "mediagovkw",
+ "https://media.gov.kw/LiveTV.aspx?PanChannel=KTVSports",
+ ),
+ ]
| media.gov.kw / mangomolo.com plugin request
### Checklist
- [X] This is a plugin request and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin requests](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+request%22)
### Description
Request to open new issue ticket from bastimeyer :
> https://media.gov.kw is now using a different stream host, namely https://mangomolo.com.
>
> This requires a separate plugin implementation and the hiplayer plugin needs to be updated accordingly with a removal of the unsupported matcher.
Initial issue :
https://github.com/streamlink/streamlink/issues/5846
### Input URLs
https://player.mangomolo.com/v1/live?id=xxxxxx
https://kwtktv1ta.cdn.mangomolo.com/ktv1/smil:ktv1.stream.smil/playlist.m3u8?xxx
| 2024-02-21T13:16:52 |
|
streamlink/streamlink | 5,854 | streamlink__streamlink-5854 | [
"5702"
] | 57dacf7bc9ee1f5793f8aa3c715220ded19653f6 | diff --git a/src/streamlink/plugins/vimeo.py b/src/streamlink/plugins/vimeo.py
--- a/src/streamlink/plugins/vimeo.py
+++ b/src/streamlink/plugins/vimeo.py
@@ -171,10 +171,12 @@ def _get_streams(self):
streams = []
+ hls = hls or {}
for url in hls.values():
streams.extend(HLSStream.parse_variant_playlist(self.session, url).items())
break
+ dash = dash or {}
for url in dash.values():
p = urlparse(url)
if p.path.endswith("dash.mpd"):
| plugins.vimeo: live event URL triggers ValidationError / DASH errors
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
6.4.2 on windows 10
### Description
A live event on vimeo fails while being launched as event or video page
### Debug log
```text
D:\_Downloads\TV+Streaming\youtubedl-streamlink\bin> .\streamlink.exe https://vimeo.com/event/3924129
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.11.6
[cli][debug] OpenSSL: OpenSSL 3.0.11 19 Sep 2023
[cli][debug] Streamlink: 6.4.2
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.11.17
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.3
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.19.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.22.2
[cli][debug] trio-websocket: 0.11.1
[cli][debug] typing-extensions: 4.8.0
[cli][debug] urllib3: 2.0.6
[cli][debug] websocket-client: 1.6.3
[cli][debug] Arguments:
[cli][debug] url=https://vimeo.com/event/3924129
[cli][debug] --loglevel=debug
[cli][debug] --player=D:\Program Files\VideoLAN\VLC\vlc.exe
[cli][debug] --player-args=--qt-minimal-view
[cli][debug] --default-stream=['720p', '720p_alt', '720p60', 'best']
[cli][debug] --retry-max=2
[cli][debug] --stream-segment-threads=3
[cli][debug] --stream-timeout=20.0
[cli][debug] --hls-playlist-reload-attempts=3
[cli][debug] --ffmpeg-ffmpeg="D:\_Downloads\TV+Streaming\youtubedl-streamlink\ffmpeg\ffmpeg.exe"
[cli][debug] --generic-playlist-max=3
[cli][debug] --generic-ignore-same-url=True
[cli][info] Found matching plugin vimeo for URL https://vimeo.com/event/3924129
[cli][error] Unable to validate response text: ValidationError(dict):
Key 'uri' not found in <{'type': 'video', 'version': '1.0', 'provider_name': 'V...>
[cli][info] Waiting for streams, retrying every 1 second(s)
[cli][error] Unable to validate response text: ValidationError(dict):
Key 'uri' not found in <{'type': 'video', 'version': '1.0', 'provider_name': 'V...>
[cli][error] Unable to validate response text: ValidationError(dict):
Key 'uri' not found in <{'type': 'video', 'version': '1.0', 'provider_name': 'V...>
error: No playable streams found on this URL: https://vimeo.com/event/3924129
=========================
D:\_Downloads\TV+Streaming\youtubedl-streamlink\bin> .\streamlink.exe https://vimeo.com/890064882
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.11.6
[cli][debug] OpenSSL: OpenSSL 3.0.11 19 Sep 2023
[cli][debug] Streamlink: 6.4.2
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.11.17
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.3
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.19.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.22.2
[cli][debug] trio-websocket: 0.11.1
[cli][debug] typing-extensions: 4.8.0
[cli][debug] urllib3: 2.0.6
[cli][debug] websocket-client: 1.6.3
[cli][debug] Arguments:
[cli][debug] url=https://vimeo.com/890064882
[cli][debug] --loglevel=debug
[cli][debug] --player=D:\Program Files\VideoLAN\VLC\vlc.exe
[cli][debug] --player-args=--qt-minimal-view
[cli][debug] --default-stream=['720p', '720p_alt', '720p60', 'best']
[cli][debug] --retry-max=2
[cli][debug] --stream-segment-threads=3
[cli][debug] --stream-timeout=20.0
[cli][debug] --hls-playlist-reload-attempts=3
[cli][debug] --ffmpeg-ffmpeg="D:\_Downloads\TV+Streaming\youtubedl-streamlink\ffmpeg\ffmpeg.exe"
[cli][debug] --generic-playlist-max=3
[cli][debug] --generic-ignore-same-url=True
[cli][info] Found matching plugin vimeo for URL https://vimeo.com/890064882
[utils.l10n][debug] Language code: en_US
Traceback (most recent call last):
File "<frozen runpy>", line 198, in _run_module_as_main
File "<frozen runpy>", line 88, in _run_code
File "D:\_Downloads\TV+Streaming\youtubedl-streamlink\bin\streamlink.exe\__main__.py", line 18, in <module>
File "D:\_Downloads\TV+Streaming\youtubedl-streamlink\pkgs\streamlink_cli\main.py", line 929, in main
handle_url()
File "D:\_Downloads\TV+Streaming\youtubedl-streamlink\pkgs\streamlink_cli\main.py", line 549, in handle_url
streams = fetch_streams_with_retry(plugin, retry_streams, retry_max)
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "D:\_Downloads\TV+Streaming\youtubedl-streamlink\pkgs\streamlink_cli\main.py", line 458, in fetch_streams_with_retry
streams = fetch_streams(plugin)
^^^^^^^^^^^^^^^^^^^^^
File "D:\_Downloads\TV+Streaming\youtubedl-streamlink\pkgs\streamlink_cli\main.py", line 449, in fetch_streams
return plugin.streams(stream_types=args.stream_types,
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "D:\_Downloads\TV+Streaming\youtubedl-streamlink\pkgs\streamlink\plugin\plugin.py", line 375, in streams
ostreams = list(ostreams)
^^^^^^^^^^^^^^
File "D:\_Downloads\TV+Streaming\youtubedl-streamlink\pkgs\streamlink\plugins\vimeo.py", line 178, in _get_streams
for url in dash.values():
^^^^^^^^^^^
AttributeError: 'NoneType' object has no attribute 'values'
```
| > [cli][debug] --generic-playlist-max=3
> [cli][debug] --generic-ignore-same-url=True
Those are not part of Streamlink... Unrelated to the plugin issue though...
It seems the issue appears just with live events somehow. Tested with an static video (not a live event) that I found at https://vimeo.com/categories/events and the plugin works.
I'll try to found a live video for testing...
```
\bin> .\streamlink.exe https://vimeo.com/737854276
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.11.6
[cli][debug] OpenSSL: OpenSSL 3.0.11 19 Sep 2023
[cli][debug] Streamlink: 6.4.2
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.11.17
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.3
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.19.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.22.2
[cli][debug] trio-websocket: 0.11.1
[cli][debug] typing-extensions: 4.8.0
[cli][debug] urllib3: 2.0.6
[cli][debug] websocket-client: 1.6.3
[cli][debug] Arguments:
[cli][debug] url=https://vimeo.com/737854276
[cli][debug] --loglevel=debug
[cli][debug] --player=D:\Program Files\VideoLAN\VLC\vlc.exe
[cli][debug] --player-args=--qt-minimal-view
[cli][debug] --default-stream=['720p', '720p_alt', '720p60', 'best']
[cli][debug] --retry-max=2
[cli][debug] --stream-segment-threads=3
[cli][debug] --stream-timeout=20.0
[cli][debug] --hls-playlist-reload-attempts=3
[cli][debug] --ffmpeg-ffmpeg="D:\_Downloads\TV+Streaming\youtubedl-streamlink\ffmpeg\ffmpeg.exe"
[cli][debug] --generic-playlist-max=3
[cli][debug] --generic-ignore-same-url=True
[cli][info] Found matching plugin vimeo for URL https://vimeo.com/737854276
[utils.l10n][debug] Language code: en_US
[stream.ffmpegmux][warning] No valid FFmpeg binary was found. See the --ffmpeg-ffmpeg option.
[stream.ffmpegmux][warning] Muxing streams is unsupported! Only a subset of the available streams can be returned!
[utils.l10n][debug] Language code: en_US
[stream.dash][debug] Available languages for DASH audio streams: NONE (using: n/a)
[cli][info] Available streams: 360p_http, 240p (worst), 240p+a66k, 240p+a98k, 240p+a128k, 240p+a192k, 360p, 360p+a66k, 360p+a98k, 360p+a128k, 360p+a192k, 540p, 540p+a66k, 540p+a98k, 540p+a128k, 540p+a192k, 720p, 720p+a66k, 720p+a98k, 720p+a128k, 720p+a192k, 1080p, 1080p+a66k, 1080p+a98k, 1080p+a128k, 1080p+a192k (best)
[cli][info] Opening stream: 720p (hls)
[cli][info] Starting player: D:\Program Files\VideoLAN\VLC\vlc.exe
[stream.hls][debug] Reloading playlist
[cli][debug] Pre-buffering 8192 bytes
[stream.hls][debug] First Sequence: 0; Last Sequence: 630
[stream.hls][debug] Start offset: 0; Duration: None; Start Sequence: 0; End Sequence: 630
[stream.hls][debug] Adding segment 0 to queue
[stream.hls][debug] Adding segment 1 to queue
[stream.hls][debug] Adding segment 2 to queue
[stream.hls][debug] Adding segment 3 to queue
[stream.hls][debug] Adding segment 4 to queue
[stream.hls][debug] Adding segment 5 to queue
[stream.hls][debug] Adding segment 6 to queue
[stream.hls][debug] Adding segment 7 to queue
[stream.hls][debug] Adding segment 8 to queue
[stream.hls][debug] Adding segment 9 to queue
[stream.hls][debug] Adding segment 10 to queue
[stream.hls][debug] Adding segment 11 to queue
[stream.hls][debug] Adding segment 12 to queue
[stream.hls][debug] Adding segment 13 to queue
[stream.hls][debug] Adding segment 14 to queue
[stream.hls][debug] Adding segment 15 to queue
[stream.hls][debug] Adding segment 16 to queue
[stream.hls][debug] Adding segment 17 to queue
[stream.hls][debug] Adding segment 18 to queue
[stream.hls][debug] Adding segment 19 to queue
[stream.hls][debug] Adding segment 20 to queue
[stream.hls][debug] Adding segment 21 to queue
[stream.hls][debug] Writing segment 0 to output
[stream.hls][debug] Segment 0 complete
[cli.output][debug] Opening subprocess: ['D:\\Program Files\\VideoLAN\\VLC\\vlc.exe', '--input-title-format', 'https://vimeo.com/737854276', '--qt-minimal-view', '-']
[stream.hls][debug] Adding segment 22 to queue
[stream.hls][debug] Writing segment 1 to output
[stream.hls][debug] Segment 1 complete
[stream.hls][debug] Adding segment 23 to queue
[cli][debug] Writing stream to output
[stream.hls][debug] Writing segment 2 to output
[stream.hls][debug] Segment 2 complete
[stream.hls][debug] Adding segment 24 to queue
[stream.hls][debug] Writing segment 3 to output
[stream.hls][debug] Segment 3 complete
...
```
It's failing even sooner for me. (Find a livestream here: https://vimeo.com/search?live=now )
```
root@pegasus:/# streamlink https://vimeo.com/908518904 --loglevel=debug
[cli][info] streamlink is running as root! Be careful!
[cli][debug] OS: Linux-6.7.1-arch1-1-x86_64-with
[cli][debug] Python: 3.11.7
[cli][debug] OpenSSL: OpenSSL 3.1.4 24 Oct 2023
[cli][debug] Streamlink: 6.5.1
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.11.17
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.4
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.20.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.21.0
[cli][debug] trio-websocket: 0.11.1
[cli][debug] typing-extensions: 4.9.0
[cli][debug] urllib3: 1.26.18
[cli][debug] websocket-client: 1.7.0
[cli][debug] Arguments:
[cli][debug] url=https://vimeo.com/908518904
[cli][debug] --loglevel=debug
[cli][info] Found matching plugin vimeo for URL https://vimeo.com/908518904
[utils.l10n][debug] Language code: en_US
Traceback (most recent call last):
File "/usr/bin/streamlink", line 8, in <module>
sys.exit(main())
^^^^^^
File "/usr/lib/python3.11/site-packages/streamlink_cli/main.py", line 934, in main
handle_url()
File "/usr/lib/python3.11/site-packages/streamlink_cli/main.py", line 556, in handle_url
streams = fetch_streams(plugin)
^^^^^^^^^^^^^^^^^^^^^
File "/usr/lib/python3.11/site-packages/streamlink_cli/main.py", line 454, in fetch_streams
return plugin.streams(stream_types=args.stream_types,
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
File "/usr/lib/python3.11/site-packages/streamlink/plugin/plugin.py", line 375, in streams
ostreams = list(ostreams)
^^^^^^^^^^^^^^
File "/usr/lib/python3.11/site-packages/streamlink/plugins/vimeo.py", line 178, in _get_streams
for url in dash.values():
^^^^^^^^^^^
AttributeError: 'NoneType' object has no attribute 'values'
```
Same error for me with a live stream. | 2024-02-22T10:35:44 |
|
streamlink/streamlink | 5,858 | streamlink__streamlink-5858 | [
"5857"
] | f205f541ed1497a0c83433e0206e6bce10a2530d | diff --git a/src/streamlink/plugins/ltv_lsm_lv.py b/src/streamlink/plugins/ltv_lsm_lv.py
--- a/src/streamlink/plugins/ltv_lsm_lv.py
+++ b/src/streamlink/plugins/ltv_lsm_lv.py
@@ -1,73 +1,42 @@
"""
$description Live TV channels from LTV, a Latvian public, state-owned broadcaster.
$url ltv.lsm.lv
-$type live
+$url replay.lsm.lv
+$type live, vod
$region Latvia
"""
import logging
import re
-from urllib.parse import urlsplit, urlunsplit
from streamlink.plugin import Plugin, pluginmatcher
from streamlink.plugin.api import validate
-from streamlink.stream.hls import HLSStream, HLSStreamReader, HLSStreamWorker
+from streamlink.stream.hls import HLSStream
log = logging.getLogger(__name__)
-def copy_query_url(to, from_):
- """
- Replace the query string in one URL with the query string from another URL
- """
- return urlunsplit(urlsplit(to)._replace(query=urlsplit(from_).query))
-
-
-class LTVHLSStreamWorker(HLSStreamWorker):
- def process_segments(self, playlist, segments):
- super().process_segments(playlist, segments)
- # update the segment URLs with the query string from the playlist URL
- for sequence in segments:
- sequence.uri = copy_query_url(sequence.uri, self.stream.url)
-
-
-class LTVHLSStreamReader(HLSStreamReader):
- __worker__ = LTVHLSStreamWorker
-
-
-class LTVHLSStream(HLSStream):
- __reader__ = LTVHLSStreamReader
-
- @classmethod
- def parse_variant_playlist(cls, *args, **kwargs):
- streams = super().parse_variant_playlist(*args, **kwargs)
-
- for stream in streams.values():
- stream.args["url"] = copy_query_url(stream.args["url"], stream.multivariant.uri)
-
- return streams
-
-
@pluginmatcher(re.compile(
- r"https://ltv\.lsm\.lv/lv/tiesraide",
+ r"https://(?:ltv|replay)\.lsm\.lv/(?:lv/tiesraide|ru/efir)/",
))
class LtvLsmLv(Plugin):
- URL_IFRAME = "https://ltv.lsm.lv/embed/live?c={embed_id}"
URL_API = "https://player.cloudycdn.services/player/ltvlive/channel/{channel_id}/"
def _get_streams(self):
self.session.http.headers.update({"Referer": self.url})
- embed_id = self.session.http.get(self.url, schema=validate.Schema(
- re.compile(r"""embed_id\s*:\s*(?P<q>")(?P<embed_id>\w+)(?P=q)"""),
- validate.any(None, validate.get("embed_id")),
+ iframe_url = self.session.http.get(self.url, schema=validate.Schema(
+ re.compile(r"""(?P<q>")https:\\u002F\\u002Fltv\.lsm\.lv\\u002Fembed\\u002Flive\?\S+?(?P=q)"""),
+ validate.none_or_all(
+ validate.get(0),
+ validate.parse_json(),
+ ),
))
- if not embed_id:
+ if not iframe_url:
+ log.error("Could not find video player iframe")
return
- log.debug(f"Found embed ID: {embed_id}")
- iframe_url = self.URL_IFRAME.format(embed_id=embed_id)
starts_at, channel_id = self.session.http.get(iframe_url, schema=validate.Schema(
validate.parse_html(),
validate.xml_xpath_string(".//live[1]/@*[name()=':embed-data']"),
@@ -112,7 +81,7 @@ def _get_streams(self):
),
)
for surl in stream_sources:
- yield from LTVHLSStream.parse_variant_playlist(self.session, surl).items()
+ yield from HLSStream.parse_variant_playlist(self.session, surl).items()
__plugin__ = LtvLsmLv
| diff --git a/tests/plugins/test_ltv_lsm_lv.py b/tests/plugins/test_ltv_lsm_lv.py
--- a/tests/plugins/test_ltv_lsm_lv.py
+++ b/tests/plugins/test_ltv_lsm_lv.py
@@ -6,20 +6,15 @@ class TestPluginCanHandleUrlLtvLsmLv(PluginCanHandleUrl):
__plugin__ = LtvLsmLv
should_match = [
- "https://ltv.lsm.lv/lv/tiesraide/example/",
- "https://ltv.lsm.lv/lv/tiesraide/example/",
- "https://ltv.lsm.lv/lv/tiesraide/example/live.123/",
- "https://ltv.lsm.lv/lv/tiesraide/example/live.123/",
- ]
-
- should_not_match = [
- "https://ltv.lsm.lv",
- "http://ltv.lsm.lv",
- "https://ltv.lsm.lv/lv",
- "http://ltv.lsm.lv/lv",
- "https://ltv.lsm.lv/other-site/",
- "http://ltv.lsm.lv/other-site/",
- "https://ltv.lsm.lv/lv/other-site/",
- "http://ltv.lsm.lv/lv/other-site/",
- "https://ltv.lsm.lv/lv/tieshraide/example/",
+ "https://ltv.lsm.lv/lv/tiesraide/ltv1",
+ "https://ltv.lsm.lv/lv/tiesraide/ltv7",
+ "https://ltv.lsm.lv/lv/tiesraide/visiem",
+ "https://ltv.lsm.lv/lv/tiesraide/lr1",
+ "https://ltv.lsm.lv/lv/tiesraide/lr2",
+ "https://ltv.lsm.lv/lv/tiesraide/lr3",
+ "https://ltv.lsm.lv/lv/tiesraide/lr4",
+ "https://ltv.lsm.lv/lv/tiesraide/lr5",
+ "https://ltv.lsm.lv/lv/tiesraide/lr6",
+ "https://replay.lsm.lv/lv/tiesraide/ltv7/sporta-studija-aizkulises",
+ "https://replay.lsm.lv/ru/efir/ltv7/sporta-studija-aizkulises",
]
| plugins.ltv_lsm_lv: No playable streams found on this URL
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
streamlink 6.6.2
### Description
Since approx. the beginning of 2024 this plugin does not extract the stream url via `--stream-url` anymore.
Following command before was working correctly and now it does not anymore.
```
streamlink --stream-url https://ltv.lsm.lv/lv/tiesraide/ltv1 best
```
### Debug log
```text
error: No playable streams found on this URL: https://ltv.lsm.lv/lv/tiesraide/ltv1
```
| 2024-02-24T09:53:21 |
|
streamlink/streamlink | 5,861 | streamlink__streamlink-5861 | [
"5856"
] | ef25765c8d7aa8e70fa36a01dacad50326948ed1 | diff --git a/src/streamlink/stream/hls/hls.py b/src/streamlink/stream/hls/hls.py
--- a/src/streamlink/stream/hls/hls.py
+++ b/src/streamlink/stream/hls/hls.py
@@ -243,9 +243,12 @@ def write(self, segment: HLSSegment, result: Response, *data):
self.reader.pause()
def _write(self, segment: HLSSegment, result: Response, is_map: bool):
- if segment.key and segment.key.method != "NONE":
+ # TODO: Rewrite HLSSegment, HLSStreamWriter and HLSStreamWorker based on independent initialization section segments,
+ # similar to the DASH implementation
+ key = segment.map.key if is_map and segment.map else segment.key
+ if key and key.method != "NONE":
try:
- decryptor = self.create_decryptor(segment.key, segment.num)
+ decryptor = self.create_decryptor(key, segment.num)
except (StreamError, ValueError) as err:
log.error(f"Failed to create decryptor: {err}")
self.close()
diff --git a/src/streamlink/stream/hls/m3u8.py b/src/streamlink/stream/hls/m3u8.py
--- a/src/streamlink/stream/hls/m3u8.py
+++ b/src/streamlink/stream/hls/m3u8.py
@@ -353,6 +353,7 @@ def parse_tag_ext_x_map(self, value: str) -> None: # version >= 5
byterange = self.parse_byterange(attr.get("BYTERANGE", ""))
self._map = Map(
uri=self.uri(uri),
+ key=self._key,
byterange=byterange,
)
diff --git a/src/streamlink/stream/hls/segment.py b/src/streamlink/stream/hls/segment.py
--- a/src/streamlink/stream/hls/segment.py
+++ b/src/streamlink/stream/hls/segment.py
@@ -46,6 +46,7 @@ class Key(NamedTuple):
# EXT-X-MAP
class Map(NamedTuple):
uri: str
+ key: Optional[Key]
byterange: Optional[ByteRange]
| diff --git a/tests/stream/hls/test_hls.py b/tests/stream/hls/test_hls.py
--- a/tests/stream/hls/test_hls.py
+++ b/tests/stream/hls/test_hls.py
@@ -1,3 +1,4 @@
+import itertools
import os
import typing
import unittest
@@ -59,7 +60,7 @@ class TagMapEnc(EncryptedBase, TagMap):
class TagKey(Tag):
- path = "encryption.key"
+ _id = itertools.count()
def __init__(self, method="NONE", uri=None, iv=None, keyformat=None, keyformatversions=None):
attrs = {"METHOD": method}
@@ -73,6 +74,7 @@ def __init__(self, method="NONE", uri=None, iv=None, keyformat=None, keyformatve
attrs["KEYFORMATVERSIONS"] = self.val_quoted_string(keyformatversions)
super().__init__("EXT-X-KEY", attrs)
self.uri = uri
+ self.path = f"encryption{next(self._id)}.key"
def url(self, namespace):
return self.uri.format(namespace=namespace) if self.uri else super().url(namespace)
@@ -718,7 +720,6 @@ def test_hls_encrypted_aes128_with_map(self):
self.mock("GET", self.url(map1), content=map1.content)
self.mock("GET", self.url(map2), content=map2.content)
- # noinspection PyTypeChecker
segments = self.subject([
Playlist(0, [key, map1] + [SegmentEnc(num, aesKey, aesIv) for num in range(2)]),
Playlist(2, [key, map2] + [SegmentEnc(num, aesKey, aesIv) for num in range(2, 4)], end=True),
@@ -732,6 +733,52 @@ def test_hls_encrypted_aes128_with_map(self):
map1, segments[0], segments[1], map2, segments[2], segments[3],
], prop="content_plain")
+ def test_hls_encrypted_aes128_with_differently_encrypted_map(self):
+ aesKey1, aesIv1, key1 = self.gen_key() # init key
+ aesKey2, aesIv2, key2 = self.gen_key() # media key
+ map1 = TagMapEnc(1, namespace=self.id(), key=aesKey1, iv=aesIv1)
+ map2 = TagMapEnc(2, namespace=self.id(), key=aesKey1, iv=aesIv1)
+ self.mock("GET", self.url(map1), content=map1.content)
+ self.mock("GET", self.url(map2), content=map2.content)
+
+ segments = self.subject([
+ Playlist(0, [key1, map1, key2] + [SegmentEnc(num, aesKey2, aesIv2) for num in range(2)]),
+ Playlist(2, [key1, map2, key2] + [SegmentEnc(num, aesKey2, aesIv2) for num in range(2, 4)], end=True),
+ ])
+
+ self.await_write(1 + 2 + 1 + 2) # 1 map, 2 segments, 1 map, 2 segments
+ data = self.await_read(read_all=True)
+ self.await_close()
+
+ assert data == self.content([
+ map1, segments[0], segments[1], map2, segments[2], segments[3],
+ ], prop="content_plain")
+
+ def test_hls_encrypted_aes128_with_plaintext_map(self):
+ aesKey, aesIv, key = self.gen_key()
+ map1 = TagMap(1, namespace=self.id())
+ map2 = TagMap(2, namespace=self.id())
+ self.mock("GET", self.url(map1), content=map1.content)
+ self.mock("GET", self.url(map2), content=map2.content)
+
+ segments = self.subject([
+ Playlist(0, [map1, key] + [SegmentEnc(num, aesKey, aesIv) for num in range(2)]),
+ Playlist(2, [map2, key] + [SegmentEnc(num, aesKey, aesIv) for num in range(2, 4)], end=True),
+ ])
+
+ self.await_write(1 + 2 + 1 + 2) # 1 map, 2 segments, 1 map, 2 segments
+ data = self.await_read(read_all=True)
+ self.await_close()
+
+ assert data == (
+ map1.content
+ + segments[0].content_plain
+ + segments[1].content_plain
+ + map2.content
+ + segments[2].content_plain
+ + segments[3].content_plain
+ )
+
def test_hls_encrypted_aes128_key_uri_override(self):
aesKey, aesIv, key = self.gen_key(uri="http://real-mocked/{namespace}/encryption.key?foo=bar")
aesKeyInvalid = bytes(ord(aesKey[i:i + 1]) ^ 0xFF for i in range(16))
| stream.hls: streamlink incorrectly decrypts media initialization section and gets error
### Checklist
- [X] This is a bug report and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed bug reports](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22bug%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
streamlink 6.6.2
### Description
I recently encountered an HLS playlist that streamlink incorrectly decrypts media initialization section and gets: `Error while decrypting segment 1: Data must be padded to 16 byte boundary in CBC mode`.
As shown in the `-l all` output (please see [this gist](https://gist.github.com/thisisgk/8c9e4a4c3ed1ccf9008a0eefd40c6c3b)), `#EXT-X-MAP:URI=...` is before `#EXT-X-KEY:METHOD=AES-128,URI=...`, so media initialization section is plain data.
Please note that this playlist might not be accessible from outside Japan and will expire in a day.
### Debug log
```text
$ streamlink -l debug --hls-duration 00:20 --http-cookie domand_bid=5e377f548bdfca20defd15f0b178b8b42e538ed14569c97f6338178cde548a49 'https://delivery.domand.nicovideo.jp/hlsbid/65d4695bd76609a88f8bfe85/playlists/media/audio-aac-192kbps.m3u8?session=8b75576e7a29181c14eb064f0359172c7d7896c56598baea0000000065da9fe6f06982f4871bf258&Expires=1708826598&Signature=r0s0nwOBDvaFFKTHFC22EuBanWTXQ0gSXQ2gDAn41bDDtAWsSb1k3yxKAO6-X96as8A2jH4pvoYiw8EKO4p704xTcRGijnmxy2fSBelLhSZ~C0KZNXnWNzgmF15zPKGc1BS8py2RF1UpYRP9y23hPbQR2eWh9c1-XEFnK~drvCltt5O8NgiR79OkZ5WzxR2mhvReS7XUoiNekR1mQsagRsTlZ-Tyktod8imWiMwPGQ6rx0zfohFliGh6Iwb~AJqLS7B4B3FWOxrsA2MTe4SxX97yoLReZ-sNZNrdwc-P5vZ8HSeiGERR4riGyNJpKEalsfG4OKHb-BoIGM2yWjjZeQ__&Key-Pair-Id=K11RB80NFXU134' best
[session][debug] Loading plugin: hls
[cli][debug] OS: macOS 10.13.6
[cli][debug] Python: 3.12.2
[cli][debug] OpenSSL: OpenSSL 1.1.1w 11 Sep 2023
[cli][debug] Streamlink: 6.6.2
[cli][debug] Dependencies:
[cli][debug] certifi: 2024.2.2
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 5.1.0
[cli][debug] pycountry: 23.12.11
[cli][debug] pycryptodome: 3.20.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.24.0
[cli][debug] trio-websocket: 0.11.1
[cli][debug] typing-extensions: 4.9.0
[cli][debug] urllib3: 2.2.1
[cli][debug] websocket-client: 1.7.0
[cli][debug] Arguments:
[cli][debug] url=https://delivery.domand.nicovideo.jp/hlsbid/65d4695bd76609a88f8bfe85/playlists/media/audio-aac-192kbps.m3u8?session=8b75576e7a29181c14eb064f0359172c7d7896c56598baea0000000065da9fe6f06982f4871bf258&Expires=1708826598&Signature=r0s0nwOBDvaFFKTHFC22EuBanWTXQ0gSXQ2gDAn41bDDtAWsSb1k3yxKAO6-X96as8A2jH4pvoYiw8EKO4p704xTcRGijnmxy2fSBelLhSZ~C0KZNXnWNzgmF15zPKGc1BS8py2RF1UpYRP9y23hPbQR2eWh9c1-XEFnK~drvCltt5O8NgiR79OkZ5WzxR2mhvReS7XUoiNekR1mQsagRsTlZ-Tyktod8imWiMwPGQ6rx0zfohFliGh6Iwb~AJqLS7B4B3FWOxrsA2MTe4SxX97yoLReZ-sNZNrdwc-P5vZ8HSeiGERR4riGyNJpKEalsfG4OKHb-BoIGM2yWjjZeQ__&Key-Pair-Id=K11RB80NFXU134
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --player=mpv
[cli][debug] --verbose-player=True
[cli][debug] --player-no-close=True
[cli][debug] --hls-duration=20.0
[cli][debug] --http-cookie=[('domand_bid', '5e377f548bdfca20defd15f0b178b8b42e538ed14569c97f6338178cde548a49')]
[cli][info] Found matching plugin hls for URL https://delivery.domand.nicovideo.jp/hlsbid/65d4695bd76609a88f8bfe85/playlists/media/audio-aac-192kbps.m3u8?session=8b75576e7a29181c14eb064f0359172c7d7896c56598baea0000000065da9fe6f06982f4871bf258&Expires=1708826598&Signature=r0s0nwOBDvaFFKTHFC22EuBanWTXQ0gSXQ2gDAn41bDDtAWsSb1k3yxKAO6-X96as8A2jH4pvoYiw8EKO4p704xTcRGijnmxy2fSBelLhSZ~C0KZNXnWNzgmF15zPKGc1BS8py2RF1UpYRP9y23hPbQR2eWh9c1-XEFnK~drvCltt5O8NgiR79OkZ5WzxR2mhvReS7XUoiNekR1mQsagRsTlZ-Tyktod8imWiMwPGQ6rx0zfohFliGh6Iwb~AJqLS7B4B3FWOxrsA2MTe4SxX97yoLReZ-sNZNrdwc-P5vZ8HSeiGERR4riGyNJpKEalsfG4OKHb-BoIGM2yWjjZeQ__&Key-Pair-Id=K11RB80NFXU134
[plugins.hls][debug] URL=https://delivery.domand.nicovideo.jp/hlsbid/65d4695bd76609a88f8bfe85/playlists/media/audio-aac-192kbps.m3u8?session=8b75576e7a29181c14eb064f0359172c7d7896c56598baea0000000065da9fe6f06982f4871bf258&Expires=1708826598&Signature=r0s0nwOBDvaFFKTHFC22EuBanWTXQ0gSXQ2gDAn41bDDtAWsSb1k3yxKAO6-X96as8A2jH4pvoYiw8EKO4p704xTcRGijnmxy2fSBelLhSZ~C0KZNXnWNzgmF15zPKGc1BS8py2RF1UpYRP9y23hPbQR2eWh9c1-XEFnK~drvCltt5O8NgiR79OkZ5WzxR2mhvReS7XUoiNekR1mQsagRsTlZ-Tyktod8imWiMwPGQ6rx0zfohFliGh6Iwb~AJqLS7B4B3FWOxrsA2MTe4SxX97yoLReZ-sNZNrdwc-P5vZ8HSeiGERR4riGyNJpKEalsfG4OKHb-BoIGM2yWjjZeQ__&Key-Pair-Id=K11RB80NFXU134; params={}
[utils.l10n][debug] Language code: en_US
[cli][info] Available streams: live (worst, best)
[cli][info] Opening stream: live (hls)
[cli][info] Starting player: mpv
[stream.hls][debug] Reloading playlist
[cli][debug] Pre-buffering 8192 bytes
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] First Sequence: 1; Last Sequence: 29
[stream.hls][debug] Start offset: 0; Duration: 20; Start Sequence: 1; End Sequence: 29
[stream.hls][debug] Adding segment 1 to queue
[stream.hls][debug] Adding segment 2 to queue
[stream.hls][debug] Adding segment 3 to queue
[stream.hls][debug] Adding segment 4 to queue
[stream.hls][info] Stopping stream early after 20
[stream.segmented][debug] Closing worker thread
[stream.hls][debug] Writing segment 1 to output
[stream.hls][error] Error while decrypting segment 1: Data must be padded to 16 byte boundary in CBC mode
[stream.hls][debug] Writing segment 1 to output
[stream.hls][debug] Segment 1 complete
[stream.hls][debug] Writing segment 2 to output
[cli.output][debug] Opening subprocess: ['/usr/local/bin/mpv', '--force-media-title=https://delivery.domand.nicovideo.jp/hlsbid/65d4695bd76609a88f8bfe85/playlists/media/audio-aac-192kbps.m3u8?session=8b75576e7a29181c14eb064f0359172c7d7896c56598baea0000000065da9fe6f06982f4871bf258&Expires=1708826598&Signature=r0s0nwOBDvaFFKTHFC22EuBanWTXQ0gSXQ2gDAn41bDDtAWsSb1k3yxKAO6-X96as8A2jH4pvoYiw8EKO4p704xTcRGijnmxy2fSBelLhSZ~C0KZNXnWNzgmF15zPKGc1BS8py2RF1UpYRP9y23hPbQR2eWh9c1-XEFnK~drvCltt5O8NgiR79OkZ5WzxR2mhvReS7XUoiNekR1mQsagRsTlZ-Tyktod8imWiMwPGQ6rx0zfohFliGh6Iwb~AJqLS7B4B3FWOxrsA2MTe4SxX97yoLReZ-sNZNrdwc-P5vZ8HSeiGERR4riGyNJpKEalsfG4OKHb-BoIGM2yWjjZeQ__&Key-Pair-Id=K11RB80NFXU134', '-']
[stream.hls][debug] Segment 2 complete
[stream.hls][debug] Writing segment 3 to output
[stream.hls][debug] Segment 3 complete
[stream.hls][debug] Writing segment 4 to output
[stream.hls][debug] Segment 4 complete
[stream.segmented][debug] Closing writer thread
[cli][debug] Writing stream to output
[file] Reading from stdin...
[ffmpeg/demuxer] mov,mp4,m4a,3gp,3g2,mj2: could not find corresponding track id 1
[ffmpeg/demuxer] mov,mp4,m4a,3gp,3g2,mj2: could not find corresponding trex (id 1)
[ffmpeg/demuxer] mov,mp4,m4a,3gp,3g2,mj2: could not find corresponding track id 0
[ffmpeg/demuxer] mov,mp4,m4a,3gp,3g2,mj2: trun track id unknown, no tfhd was found
[ffmpeg/demuxer] mov,mp4,m4a,3gp,3g2,mj2: error reading header
[lavf] avformat_open_input() failed
[ffmpeg/demuxer] mov,mp4,m4a,3gp,3g2,mj2: could not find corresponding track id 1
[ffmpeg/demuxer] mov,mp4,m4a,3gp,3g2,mj2: could not find corresponding trex (id 1)
[ffmpeg/demuxer] mov,mp4,m4a,3gp,3g2,mj2: could not find corresponding track id 0
[ffmpeg/demuxer] mov,mp4,m4a,3gp,3g2,mj2: trun track id unknown, no tfhd was found
[ffmpeg/demuxer] mov,mp4,m4a,3gp,3g2,mj2: error reading header
[lavf] avformat_open_input() failed
Failed to recognize file format.
Exiting... (Errors when loading file)
[cli][info] Player closed
[cli][info] Stream ended
[cli][info] Closing currently open stream...
$
```
| Thanks for the bug report.
The issue is this:
The data of both `EXT-X-MAP` and `EXT-X-KEY` are applied to each [`HLSSegment`](https://github.com/streamlink/streamlink/blob/6.6.2/src/streamlink/stream/hls/segment.py#L99-L106) item. This is obviously the wrong choice of data structure in this special case here where the initialization section is not encrypted. The `HLSStreamWorker` will [always assume that the initialization section is encrypted](https://github.com/streamlink/streamlink/blob/6.6.2/src/streamlink/stream/hls/hls.py#L245-L246), even if the decryption key was set after the initialization section.
Fixing this issue will very likely require a lot of refactoring of the `M3U8Parser`, the `HLSStreamWorker` and `HLSStreamWriter`.
The DASH implementation for example - while of course completely different - wouldn't have this kind of issue because [there's a clear distinction between initialization section segments and regular media segments](https://github.com/streamlink/streamlink/blob/6.6.2/src/streamlink/stream/dash/segment.py#L25). The HLS implementation never made this segment-type distinction and simply added the map attribute to segments.
The whole HLS media initialization section was also very much flawed in a different way until last year, because there was a bit of confusion with no clear answer when it was implemented first (due to MPEG-TS streams). Initialization sections were applied to each segment in the output, which is of course wrong. Demuxers however skipped this redundant data, so it was never a real problem apart from warning messages. As said, this was fixed last year, but the old implementation was pretty much kept. This issue here now justifies a full rewrite based on how the DASH implementation handles initialization sections, namely as separate segments that are yielded by the worker thread. This way, initialization sections can have independent `key` attributes which are not tied to the media segments that follow.
I don't see a reason to fix this issue here based on the current implementation, so a bug fix will have to wait until the rewrite has been completed, which as said, will be a lot of work. | 2024-02-24T17:05:37 |
streamlink/streamlink | 5,869 | streamlink__streamlink-5869 | [
"5868"
] | e077e2fccdb69a3bc8bfe979b320783568f6ffab | diff --git a/src/streamlink/plugins/bloomberg.py b/src/streamlink/plugins/bloomberg.py
--- a/src/streamlink/plugins/bloomberg.py
+++ b/src/streamlink/plugins/bloomberg.py
@@ -16,14 +16,14 @@
log = logging.getLogger(__name__)
-@pluginmatcher(re.compile(r"""
- https?://(?:www\.)?bloomberg\.com/
- (?:
- (?P<live>live)(?:/(?P<channel>[^/]+))?
- |
- news/videos/[^/]+/[^/]+
- )
-""", re.VERBOSE))
+@pluginmatcher(
+ name="live",
+ pattern=re.compile(r"https?://(?:www\.)?bloomberg\.com/live(?:/(?P<channel>[^/]+))?"),
+)
+@pluginmatcher(
+ name="vod",
+ pattern=re.compile(r"https?://(?:www\.)?bloomberg\.com/news/videos/[^/]+/[^/]+"),
+)
class Bloomberg(Plugin):
LIVE_API_URL = "https://cdn.gotraffic.net/projector/latest/assets/config/config.min.json?v=1"
VOD_API_URL = "https://www.bloomberg.com/api/embed?id={0}"
@@ -106,21 +106,23 @@ def _get_vod_streams(self, data):
def _get_streams(self):
del self.session.http.headers["Accept-Encoding"]
- try:
- data = self.session.http.get(self.url, schema=validate.Schema(
- validate.parse_html(),
- validate.xml_xpath_string(".//script[contains(text(),'window.__PRELOADED_STATE__')][1]/text()"),
- str,
- validate.regex(re.compile(r"^\s*window\.__PRELOADED_STATE__\s*=\s*({.+})\s*;?\s*$", re.DOTALL)),
- validate.get(1),
- validate.parse_json(),
- ))
- except PluginError:
+ data = self.session.http.get(self.url, schema=validate.Schema(
+ validate.parse_html(),
+ validate.xml_xpath_string(".//script[contains(text(),'window.__PRELOADED_STATE__')][1]/text()"),
+ validate.none_or_all(
+ re.compile(r"\bwindow\.__PRELOADED_STATE__\s*=\s*(?P<json>{.+?})\s*;(?:\s|$)"),
+ validate.none_or_all(
+ validate.get("json"),
+ validate.parse_json(),
+ ),
+ ),
+ ))
+ if not data:
log.error("Could not find JSON data. Invalid URL or bot protection...")
return
- if self.match.group("live"):
- streams = self._get_live_streams(data, self.match.group("channel") or self.DEFAULT_CHANNEL)
+ if self.matches["live"]:
+ streams = self._get_live_streams(data, self.match["channel"] or self.DEFAULT_CHANNEL)
else:
streams = self._get_vod_streams(data)
| diff --git a/tests/plugins/test_bloomberg.py b/tests/plugins/test_bloomberg.py
--- a/tests/plugins/test_bloomberg.py
+++ b/tests/plugins/test_bloomberg.py
@@ -6,11 +6,22 @@ class TestPluginCanHandleUrlBloomberg(PluginCanHandleUrl):
__plugin__ = Bloomberg
should_match_groups = [
- ("https://www.bloomberg.com/live", {"live": "live"}),
- ("https://www.bloomberg.com/live/", {"live": "live"}),
- ("https://www.bloomberg.com/live/europe", {"live": "live", "channel": "europe"}),
- ("https://www.bloomberg.com/live/europe/", {"live": "live", "channel": "europe"}),
- ("https://www.bloomberg.com/news/videos/2022-08-10/-bloomberg-surveillance-early-edition-full-08-10-22", {}),
+ (
+ ("live", "https://www.bloomberg.com/live"),
+ {},
+ ),
+ (
+ ("live", "https://www.bloomberg.com/live/europe"),
+ {"channel": "europe"},
+ ),
+ (
+ ("live", "https://www.bloomberg.com/live/us"),
+ {"channel": "us"},
+ ),
+ (
+ ("vod", "https://www.bloomberg.com/news/videos/2022-08-10/-bloomberg-surveillance-early-edition-full-08-10-22"),
+ {},
+ ),
]
should_not_match = [
| plugins.bloomberg: error: unmatched '{' in format spec
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
streamlink 6.6.2
### Description
It's quite a strange error. Seems like there is a change to the JSON data return from Bloomberg, or it is corrupted.
### Debug log
```text
$ streamlink --loglevel=debug https://www.bloomberg.com/live/us
[session][debug] Loading plugin: bloomberg
[cli][debug] OS: macOS 10.16
[cli][debug] Python: 3.9.12
[cli][debug] OpenSSL: OpenSSL 1.1.1n 15 Mar 2022
[cli][debug] Streamlink: 6.6.2
[cli][debug] Dependencies:
[cli][debug] certifi: 2021.10.8
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.8.0
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.19.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.27.1
[cli][debug] trio: 0.22.2
[cli][debug] trio-websocket: 0.11.1
[cli][debug] typing-extensions: 4.1.1
[cli][debug] urllib3: 1.26.9
[cli][debug] websocket-client: 1.6.3
[cli][debug] Arguments:
[cli][debug] url=https://www.bloomberg.com/live/us
[cli][debug] --loglevel=debug
[cli][info] Found matching plugin bloomberg for URL https://www.bloomberg.com/live/us
error: unmatched '{' in format spec
```
| 2024-02-28T03:18:59 |
|
streamlink/streamlink | 5,881 | streamlink__streamlink-5881 | [
"5431"
] | 70d5d4a1085412a382efcaabe1cebac7687c5ff4 | diff --git a/src/streamlink/plugins/ustvnow.py b/src/streamlink/plugins/ustvnow.py
--- a/src/streamlink/plugins/ustvnow.py
+++ b/src/streamlink/plugins/ustvnow.py
@@ -21,7 +21,7 @@
@pluginmatcher(re.compile(
- r"https?://(?:www\.)?ustvnow\.com/live/(?P<scode>\w+)/-(?P<id>\d+)",
+ r"https?://(?:www\.)?ustvnow\.com/channel/live/(?P<chname>\w+)",
))
@pluginargument(
"username",
@@ -96,11 +96,6 @@ def box_id(self):
return self.cache.get("box_id")
def get_token(self):
- """
- Get the token for USTVNow
- :return: a valid token
- """
-
if not self._token:
log.debug("Getting new session token")
res = self.session.http.get(self._token_url, params={
@@ -114,13 +109,13 @@ def get_token(self):
})
data = res.json()
- if data["status"]:
- self._token = data["response"]["sessionId"]
- log.debug("New token: {}".format(self._token))
- else:
+ if not data["status"]:
log.error("Token acquisition failed: {details} ({detail})".format(**data["error"]))
raise PluginError("could not obtain token")
+ self._token = data["response"]["sessionId"]
+ log.debug(f"New token: {self._token}")
+
return self._token
def api_request(self, path, data, metadata=None):
@@ -153,21 +148,20 @@ def login(self, username, password):
return resp["data"]["status"]
def _get_streams(self):
- """
- Finds the streams from ustvnow.com.
- """
- if self.login(self.get_option("username"), self.get_option("password")):
- path = urlparse(self.url).path.strip("/")
- resp = self.api_request("send", {"path": path}, {"request": "page/stream"})
- if resp["data"]["status"]:
- for stream in resp["data"]["response"]["streams"]:
- if stream["keys"]["licenseKey"]:
- log.warning("Stream possibly protected by DRM")
- yield from HLSStream.parse_variant_playlist(self.session, stream["url"]).items()
- else:
- log.error("Could not find any streams: {code}: {message}".format(**resp["data"]["error"]))
- else:
+ if not self.login(self.get_option("username"), self.get_option("password")):
log.error("Failed to login, check username and password")
+ return
+
+ path = urlparse(self.url).path.strip("/")
+ resp = self.api_request("send", {"path": path}, {"request": "page/stream"})
+ if not resp["data"]["status"]:
+ log.error("Could not find any streams: {code}: {message}".format(**resp["data"]["error"]))
+ return
+
+ for stream in resp["data"]["response"]["streams"]:
+ if stream["keys"]["licenseKey"]:
+ log.warning("Stream possibly protected by DRM")
+ yield from HLSStream.parse_variant_playlist(self.session, stream["url"]).items()
__plugin__ = USTVNow
| diff --git a/tests/plugins/test_ustvnow.py b/tests/plugins/test_ustvnow.py
--- a/tests/plugins/test_ustvnow.py
+++ b/tests/plugins/test_ustvnow.py
@@ -6,7 +6,7 @@ class TestPluginCanHandleUrlUSTVNow(PluginCanHandleUrl):
__plugin__ = USTVNow
should_match = [
- "http://www.ustvnow.com/live/foo/-65",
+ "https://www.ustvnow.com/channel/live/cbs",
]
| plugins.ustvnow: pluginmatcher URL update needed
### Checklist
- [X] This is a plugin issue and not a different kind of issue
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
Latest build from the master branch
### Description
You need to replace the search template in ustvnow.py plugin with the one below.
```
@pluginmatcher(re.compile(
r"https?://(?:www\.)?ustvnow\.com/channel/live/(?P<chname>\w+)",
))
```
What is in the code now - does not work due to changes that have occurred on the site www.ustvnow.com
### Debug log
```text
The log cannot be retrieved because changes must be made to the template of the link it "serves"
```
| Is this issue being worked on? Its been over half a year.
Yes, I use it exactly with the changes I made and published on GitHub... I can give you the log if you need it.
1 ΠΌΠ°ΡΡΠ° 2024, 04:32:14, ΠΎΡ "MajinLugia" ***@***.***>:
Is this issue being worked on? Its been over half a year.
β
Reply to this email directly, view it on GitHub, or unsubscribe.
You are receiving this because you authored the thread.Message ID: ***@***.***>
--=-2v/3N8OZEl8eGrYBGi6O
Content-Type: text/html; charset="UTF-8"
Content-Transfer-Encoding: base64
PGh0bWw+PGJvZHk+PHNwYW4gc3R5bGU9ImRpc3BsYXk6YmxvY2s7IiBjbGFzcz0ieGZtXzExNzE5
NTM1Ij48ZGl2PjxzcGFuIHN0eWxlPSJmb250LWZhbWlseTpBcmlhbDtmb250LXNpemU6MTJwdDts
aW5lLWhlaWdodDoxNHB0OyI+WWVzLCBJIHVzZSBpdCBleGFjdGx5IHdpdGggdGhlIGNoYW5nZXMg
SSBtYWRlIGFuZCBwdWJsaXNoZWQgb24gR2l0SHViLi4uIEkgY2FuIGdpdmUgeW91IHRoZSBsb2cg
aWYgeW91IG5lZWQgaXQuIDwvc3Bhbj48L2Rpdj4NCjxkaXY+PGJyLz48L2Rpdj4NCjxkaXY+PGk+
PHNwYW4gc3R5bGU9ImZvbnQtc2l6ZToxMHB0O2xpbmUtaGVpZ2h0OjEycHQ7Ij48c3BhbiBzdHls
ZT0iZm9udC1mYW1pbHk6QXJpYWw7Ij4xINC80LDRgNGC0LAgMjAyNCwgMDQ6MzI6MTQsINC+0YIN
CiAgICAiTWFqaW5MdWdpYSIgJmx0Ozwvc3Bhbj48YSBocmVmPSJtYWlsdG86bm90aWZpY2F0aW9u
c0BnaXRodWIuY29tIiB0YXJnZXQ9Il9ibGFuayI+PHNwYW4gc3R5bGU9ImZvbnQtZmFtaWx5OkFy
aWFsOyI+bm90aWZpY2F0aW9uc0BnaXRodWIuY29tPC9zcGFuPjwvYT48c3BhbiBzdHlsZT0iZm9u
dC1mYW1pbHk6QXJpYWw7Ij4mZ3Q7Og0KPC9zcGFuPjwvc3Bhbj48L2k+PC9kaXY+DQo8ZGl2Pjxi
ci8+PC9kaXY+DQo8YmxvY2txdW90ZSBzdHlsZT0iYm9yZGVyLWxlZnQ6MXB4IHNvbGlkICNjY2Nj
Y2M7bWFyZ2luOjBweCAwcHggMHB4IDAuOGV4O3BhZGRpbmctbGVmdDoxZXg7Ij4NCjxkaXYgc3R5
bGU9ImRpc3BsYXk6YmxvY2s7Ij4NCjxkaXY+PC9kaXY+DQo8ZGl2PklzIHRoaXMgaXNzdWUgYmVp
bmcgd29ya2VkIG9uPyBJdHMgYmVlbiBvdmVyIGhhbGYgYSB5ZWFyLjwvZGl2Pg0KPGRpdj48c3Bh
biBzdHlsZT0iZm9udC1zaXplOnNtYWxsO2NvbG9yOiM2NjY7Ij7igJQ8YnIvPlJlcGx5IHRvIHRo
aXMgZW1haWwgZGlyZWN0bHksIDxhIGhyZWY9Imh0dHBzOi8vZ2l0aHViLmNvbS9zdHJlYW1saW5r
L3N0cmVhbWxpbmsvaXNzdWVzLzU0MzEjaXNzdWVjb21tZW50LTE5NzIzNDc3ODEiIHRhcmdldD0i
X2JsYW5rIiByZWw9Im5vcmVmZXJyZXIgbm9vcGVuZXIiPnZpZXcgaXQgb24gR2l0SHViPC9hPiwg
b3IgPGEgaHJlZj0iaHR0cHM6Ly9naXRodWIuY29tL25vdGlmaWNhdGlvbnMvdW5zdWJzY3JpYmUt
YXV0aC9BRU1UQUlMRUlRU1NBQUJCTzZJSEVOM1lWN1NDM0FWQ05GU002QUFBQUFBMkZMVzdCV1ZI
STJEU01WUVdJWDNMTVY0M09TTFRPTjJXS1EzUE5WV1dLM1RVSE1ZVFNOWlNHTTJET05aWUdFIiB0
YXJnZXQ9Il9ibGFuayIgcmVsPSJub3JlZmVycmVyIG5vb3BlbmVyIj51bnN1YnNjcmliZTwvYT4u
PGJyLz5Zb3UgYXJlIHJlY2VpdmluZyB0aGlzIGJlY2F1c2UgeW91IGF1dGhvcmVkIHRoZSB0aHJl
YWQuPGltZyBzcmM9Imh0dHBzOi8vZ2l0aHViLmNvbS9ub3RpZmljYXRpb25zL2JlYWNvbi9BRU1U
QUlPSEwzV0E2NlEyVlNXVVRETFlWN1NDM0E1Q05GU002QUFBQUFBMkZMVzdCV1dHRzMzTk5WU1c0
NUM3T1I0WEFaTk1KRlpYRzVMRklOWFcyM0xGTloyS1VZM1BOVldXSzNUVUw1VVdKVFRWUjZSWUsu
Z2lmIiBoZWlnaHQ9IjEiIHdpZHRoPSIxIiBhbHQ9IiIvPjwvc3Bhbj48c3BhbiBzdHlsZT0iZm9u
dC1zaXplOjA7Y29sb3I6dHJhbnNwYXJlbnQ7ZGlzcGxheTpub25lO292ZXJmbG93OmhpZGRlbjt3
aWR0aDowO2hlaWdodDowO21heC13aWR0aDowO21heC1oZWlnaHQ6MDsiPk1lc3NhZ2UgSUQ6ICZs
dDtzdHJlYW1saW5rL3N0cmVhbWxpbmsvaXNzdWVzLzU0MzEvMTk3MjM0Nzc4MUBnaXRodWIuY29t
Jmd0Ozwvc3Bhbj48L2Rpdj4NCjwvZGl2Pg0KPC9ibG9ja3F1b3RlPjwvc3Bhbj48L2JvZHk+PC9o
dG1sPg0K
--=-2v/3N8OZEl8eGrYBGi6O--
[streamlinkproxy][info] Request from: 127.0.0.1 - GET /https://www.ustvnow.com/channel/live/best_ever_tv?ustvnow-username=XXXX@XXXt&ustvnow-password=XXXX HTTP/1.1 200 -
[plugins.ustvnow][debug] Trying to login...
[plugins.ustvnow][debug] Getting new session token
[plugins.ustvnow][debug] New token: 5f175fe2-5b3c-42a5-8b63-18d9ad45506a
[utils.l10n][debug] Language code: en_GB
[streamlinkproxy][debug] USTVNOW plugin found sreams: ['216p', '360p', '540p', '720p', '1080p']
[stream.hls][debug] Reloading playlist
[stream.hls][debug] First Sequence: 3797465; Last Sequence: 3797470
[stream.hls][debug] Start offset: 0; Duration: None; Start Sequence: 3797468; End Sequence: None
[stream.hls][debug] Adding segment 3797468 to queue
[stream.hls][debug] Adding segment 3797469 to queue
[stream.hls][debug] Adding segment 3797470 to queue
[stream.hls][debug] Writing segment 3797468 to output
[stream.hls][debug] Segment 3797468 complete
[stream.hls][debug] Writing segment 3797469 to output
[stream.hls][debug] Segment 3797469 complete
[stream.hls][debug] Writing segment 3797470 to output
[stream.hls][debug] Segment 3797470 complete
[stream.hls][debug] Reloading playlist
If you want this fixed, then please open a pull request with the necessary changes and provide a full debug log without using third party tools, so we can see that you're running Streamlink from the commit of the pull request. This site requires a paid account, so this obviously won't fix itself.
- https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#pull-requests
- https://streamlink.github.io/developing.html | 2024-03-08T16:45:00 |
streamlink/streamlink | 5,890 | streamlink__streamlink-5890 | [
"5889"
] | 251fe08f8da8214dafdf094afd80c543b8e2e0fe | diff --git a/src/streamlink/plugins/tv3cat.py b/src/streamlink/plugins/tv3cat.py
--- a/src/streamlink/plugins/tv3cat.py
+++ b/src/streamlink/plugins/tv3cat.py
@@ -8,49 +8,75 @@
import logging
import re
+from streamlink.exceptions import NoStreamsError, PluginError
from streamlink.plugin import Plugin, pluginmatcher
from streamlink.plugin.api import validate
+from streamlink.stream.dash import DASHStream
from streamlink.stream.hls import HLSStream
+from streamlink.stream.http import HTTPStream
log = logging.getLogger(__name__)
-@pluginmatcher(re.compile(
- r"https?://(?:www\.)?ccma\.cat/tv3/directe/(?P<ident>.+?)/",
-))
+@pluginmatcher(
+ name="live",
+ pattern=re.compile(r"https://(?:www)?\.ccma\.cat/3cat/directes/(?P<ident>[^/?]+)"),
+)
+@pluginmatcher(
+ name="vod",
+ pattern=re.compile(r"https://(?:www)?\.ccma\.cat/3cat/[^/]+/video/(?P<ident>\d+)"),
+)
class TV3Cat(Plugin):
- _URL_STREAM_INFO = "https://dinamics.ccma.cat/pvideo/media.jsp"
+ _URL_API_GEO = "https://dinamics.ccma.cat/geo.json"
+ _URL_API_MEDIA = "https://api-media.ccma.cat/pvideo/media.jsp"
- _MAP_CHANNELS = {
- "tv3": "tvi",
+ _MAP_CHANNEL_IDENTS = {
+ "catalunya-radio": "cr",
+ "catalunya-informacio": "ci",
+ "catalunya-musica": "cm",
+ "icat": "ic",
}
- def _get_streams(self):
- ident = self.match.group("ident")
+ def _call_api_media(self, fmt, schema, params):
+ geo = self.session.http.get(
+ self._URL_API_GEO,
+ schema=validate.Schema(
+ validate.parse_json(),
+ {"geo": str},
+ validate.get("geo"),
+ ),
+ )
+ if not geo:
+ raise PluginError("Missing 'geo' value")
- schema_media = {
- "geo": str,
- "url": validate.url(path=validate.endswith(".m3u8")),
- }
+ log.debug(f"{geo=}")
+ schema = validate.all(
+ {
+ "geo": str,
+ "format": fmt,
+ "url": schema,
+ },
+ validate.union_get("geo", "url"),
+ )
- stream_infos = self.session.http.get(
- self._URL_STREAM_INFO,
+ ident = self.match["ident"]
+ streams = self.session.http.get(
+ self._URL_API_MEDIA,
params={
"media": "video",
"versio": "vast",
- "idint": self._MAP_CHANNELS.get(ident, ident),
- "profile": "pc",
- "desplacament": "0",
- "broadcast": "false",
+ "idint": self._MAP_CHANNEL_IDENTS.get(ident, ident),
+ "profile": "pc_3cat",
+ **(params or {}),
},
schema=validate.Schema(
validate.parse_json(),
{
"media": validate.any(
- [schema_media],
+ [schema],
validate.all(
- schema_media,
+ schema,
validate.transform(lambda item: [item]),
),
),
@@ -59,12 +85,41 @@ def _get_streams(self):
),
)
- for stream in stream_infos:
- log.info(f"Accessing stream from region {stream['geo']}")
- try:
- return HLSStream.parse_variant_playlist(self.session, stream["url"], name_fmt="{pixels}_{bitrate}")
- except OSError:
- pass
+ log.debug(f"{streams=}")
+ for _geo, data in streams:
+ if _geo == geo:
+ return data
+
+ log.error("The content is geo-blocked")
+ raise NoStreamsError
+
+ def _get_live(self):
+ schema = validate.url(path=validate.endswith(".m3u8"))
+ url = self._call_api_media("HLS", schema, {"desplacament": 0})
+ return HLSStream.parse_variant_playlist(self.session, url)
+
+ def _get_vod(self):
+ schema = [
+ validate.all(
+ {
+ "label": str,
+ "file": validate.url(),
+ },
+ validate.union_get("label", "file"),
+ ),
+ ]
+ urls = self._call_api_media("MP4", schema, {"format": "dm"})
+ for label, url in urls:
+ if label == "DASH":
+ yield from DASHStream.parse_manifest(self.session, url).items()
+ else:
+ yield label, HTTPStream(self.session, url)
+
+ def _get_streams(self):
+ if self.matches["live"]:
+ return self._get_live()
+ else:
+ return self._get_vod()
__plugin__ = TV3Cat
| diff --git a/tests/plugins/test_tv3cat.py b/tests/plugins/test_tv3cat.py
--- a/tests/plugins/test_tv3cat.py
+++ b/tests/plugins/test_tv3cat.py
@@ -6,8 +6,22 @@ class TestPluginCanHandleUrlTV3Cat(PluginCanHandleUrl):
__plugin__ = TV3Cat
should_match_groups = [
- ("https://ccma.cat/tv3/directe/tv3/", {"ident": "tv3"}),
- ("https://ccma.cat/tv3/directe/324/", {"ident": "324"}),
- ("https://www.ccma.cat/tv3/directe/tv3/", {"ident": "tv3"}),
- ("https://www.ccma.cat/tv3/directe/324/", {"ident": "324"}),
+ (("live", "https://www.ccma.cat/3cat/directes/tv3/"), {"ident": "tv3"}),
+ (("live", "https://www.ccma.cat/3cat/directes/324/"), {"ident": "324"}),
+ (("live", "https://www.ccma.cat/3cat/directes/esport3/"), {"ident": "esport3"}),
+ (("live", "https://www.ccma.cat/3cat/directes/sx3/"), {"ident": "sx3"}),
+ (("live", "https://www.ccma.cat/3cat/directes/catalunya-radio/"), {"ident": "catalunya-radio"}),
+
+ (
+ ("vod", "https://www.ccma.cat/3cat/t1xc1-arribada/video/6260741/"),
+ {"ident": "6260741"},
+ ),
+ (
+ ("vod", "https://www.ccma.cat/3cat/merli-els-peripatetics-capitol-1/video/5549976/"),
+ {"ident": "5549976"},
+ ),
+ (
+ ("vod", "https://www.ccma.cat/3cat/buscant-la-sostenibilitat-i-la-tecnologia-del-futur/video/6268863/"),
+ {"ident": "6268863"},
+ ),
]
| plugins.tv3cat: URLs changed
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
6.7.0
### Description
https://www.ccma.cat/3cat/directes/tv3/ is new URL of TV3CAT.
### Debug log
```text
H:\101tv>streamlink --loglevel=debug https://www.ccma.cat/3cat/directes/tv3/ best
[cli][debug] OS: Windows 11
[cli][debug] Python: 3.12.2
[cli][debug] OpenSSL: OpenSSL 3.0.13 30 Jan 2024
[cli][debug] Streamlink: 6.7.0
[cli][debug] Dependencies:
[cli][debug] certifi: 2024.2.2
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 5.1.0
[cli][debug] pycountry: 23.12.11
[cli][debug] pycryptodome: 3.20.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.24.0
[cli][debug] trio-websocket: 0.11.1
[cli][debug] typing-extensions: 4.10.0
[cli][debug] urllib3: 2.2.1
[cli][debug] websocket-client: 1.7.0
[cli][debug] Arguments:
[cli][debug] url=https://www.ccma.cat/3cat/directes/tv3/
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --ffmpeg-ffmpeg=C:\Program Files\Streamlink\ffmpeg\ffmpeg.exe
[cli][info] Found matching plugin generic for URL https://www.ccma.cat/3cat/directes/tv3/
[plugins.generic][info] Version 2023-08-24 - https://github.com/back-to/generic
[plugins.generic][info] 1. URL=https://www.ccma.cat/3cat/directes/tv3/
error: No plugin can handle URL: https://www.ccma.cat/3cat/directes/tv3/
```
| 2024-03-13T20:03:53 |
|
streamlink/streamlink | 5,908 | streamlink__streamlink-5908 | [
"5907"
] | 33879e284c7987c81cce11b3ea06fd99533c395d | diff --git a/src/streamlink/plugins/vkplay.py b/src/streamlink/plugins/vkplay.py
--- a/src/streamlink/plugins/vkplay.py
+++ b/src/streamlink/plugins/vkplay.py
@@ -1,6 +1,6 @@
"""
$description Russian live-streaming platform for gaming and esports, owned by VKontakte.
-$url vkplay.live
+$url live.vkplay.ru
$type live
$metadata id
$metadata author
@@ -20,13 +20,13 @@
@pluginmatcher(re.compile(
- r"https?://vkplay\.live/(?P<channel_name>\w+)/?$",
+ r"https?://(?:live\.vkplay\.ru|vkplay\.live)/(?P<channel_name>\w+)/?$",
))
class VKplay(Plugin):
- API_URL = "https://api.vkplay.live/v1"
+ API_URL = "https://api.live.vkplay.ru/v1"
def _get_streams(self):
- self.author = self.match.group("channel_name")
+ self.author = self.match["channel_name"]
log.debug(f"Channel name: {self.author}")
data = self.session.http.get(
| diff --git a/tests/plugins/test_vkplay.py b/tests/plugins/test_vkplay.py
--- a/tests/plugins/test_vkplay.py
+++ b/tests/plugins/test_vkplay.py
@@ -6,10 +6,12 @@ class TestPluginCanHandleUrlVKplay(PluginCanHandleUrl):
__plugin__ = VKplay
should_match = [
- "https://vkplay.live/Channel_Name_123",
+ "https://live.vkplay.ru/CHANNEL",
+ "https://vkplay.live/CHANNEL",
]
should_not_match = [
+ "https://live.vkplay.ru/",
"https://vkplay.live/",
"https://support.vkplay.ru/vkp_live",
"https://vkplay.live/app/settings/edit",
| plugins.vkplay: vkplay.live has moved to another domain (live.vkplay.ru)
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
streamlink 6.7.2
### Description
https://vk.com/wall-212496568_91026
yesterday, vkplay live changed its domain. if you specify the old domain in the link to the stream, then everything still works, but on the site itself there are links to a new domain, to which the existing plugin does not respond.
I just tried to change the updated part of the domain in the plugin code (vkplay.live -> live.vkplay.ru ), and everything seems to be working well. It's a bit difficult for me to create a pull request, but here's the corrected plugin on gist:
https://gist.github.com/oexlkinq/eef0a260dddad473c5febafd91b980d9
the old domain is also listed in the documentation (https://streamlink.github.io/plugins.html#vkplay)
### Debug log
```text
streamlink https://live.vkplay.ru/ruwarface 720p --loglevel=debug
[cli][debug] OS: Linux-6.8.1-arch1-1-x86_64-with-glibc2.39
[cli][debug] Python: 3.11.8
[cli][debug] OpenSSL: OpenSSL 3.2.1 30 Jan 2024
[cli][debug] Streamlink: 6.7.2
[cli][debug] Dependencies:
[cli][debug] certifi: 2024.2.2
[cli][debug] exceptiongroup: 1.2.0
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 5.1.0
[cli][debug] pycountry: 23.12.11
[cli][debug] pycryptodome: 3.20.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.25.0
[cli][debug] trio-websocket: 0.11.1
[cli][debug] typing-extensions: 4.10.0
[cli][debug] urllib3: 1.26.18
[cli][debug] websocket-client: 1.7.0
[cli][debug] Arguments:
[cli][debug] url=https://live.vkplay.ru/ruwarface
[cli][debug] stream=['720p']
[cli][debug] --loglevel=debug
error: No plugin can handle URL: https://live.vkplay.ru/ruwarface
```
| 2024-03-28T17:35:10 |
|
streamlink/streamlink | 5,910 | streamlink__streamlink-5910 | [
"5752"
] | 39961a597e6af4d2084903b4420590de588c139f | diff --git a/src/streamlink/plugins/pluto.py b/src/streamlink/plugins/pluto.py
--- a/src/streamlink/plugins/pluto.py
+++ b/src/streamlink/plugins/pluto.py
@@ -13,8 +13,9 @@
from urllib.parse import parse_qsl, urljoin
from uuid import uuid4
+from streamlink.exceptions import PluginError
from streamlink.plugin import Plugin, pluginmatcher
-from streamlink.plugin.api import validate
+from streamlink.plugin.api import useragents, validate
from streamlink.stream.hls import HLSStream, HLSStreamReader, HLSStreamWriter
from streamlink.utils.url import update_qsd
@@ -23,7 +24,7 @@
class PlutoHLSStreamWriter(HLSStreamWriter):
- ad_re = re.compile(r"_ad/creative/|dai\.google\.com|Pluto_TV_OandO/.*(Bumper|plutotv_filler)")
+ ad_re = re.compile(r"_ad/creative/|creative/\d+_ad/|dai\.google\.com|Pluto_TV_OandO/.*(Bumper|plutotv_filler)")
def should_filter_segment(self, segment):
return self.ad_re.search(segment.uri) is not None or super().should_filter_segment(segment)
@@ -38,152 +39,192 @@ class PlutoHLSStream(HLSStream):
__reader__ = PlutoHLSStreamReader
-@pluginmatcher(re.compile(r"""
- https?://(?:www\.)?pluto\.tv/(?:\w{2}/)?(?:
- live-tv/(?P<slug_live>[^/]+)
- |
- on-demand/series/(?P<slug_series>[^/]+)(?:/season/\d+)?/episode/(?P<slug_episode>[^/]+)
- |
- on-demand/movies/(?P<slug_movies>[^/]+)
- )/?$
-""", re.VERBOSE))
+@pluginmatcher(
+ name="live",
+ pattern=re.compile(
+ r"https?://(?:www\.)?pluto\.tv/(?:\w{2}/)?live-tv/(?P<id>[^/]+)/?$",
+ ),
+)
+@pluginmatcher(
+ name="series",
+ pattern=re.compile(
+ r"https?://(?:www\.)?pluto\.tv/(?:\w{2}/)?on-demand/series/(?P<id_s>[^/]+)(?:/season/\d+)?/episode/(?P<id_e>[^/]+)/?$",
+ ),
+)
+@pluginmatcher(
+ name="movies",
+ pattern=re.compile(
+ r"https?://(?:www\.)?pluto\.tv/(?:\w{2}/)?on-demand/movies/(?P<id>[^/]+)/?$",
+ ),
+)
class Pluto(Plugin):
- def _get_api_data(self, kind, slug, slugfilter=None):
- log.debug(f"slug={slug}")
- app_version = self.session.http.get(self.url, schema=validate.Schema(
- validate.parse_html(),
- validate.xml_xpath_string(".//head/meta[@name='appVersion']/@content"),
- validate.any(None, str),
- ))
- if not app_version:
- return
-
- log.debug(f"app_version={app_version}")
+ def __init__(self, *args, **kwargs):
+ super().__init__(*args, **kwargs)
+ self.session.http.headers.update({"User-Agent": useragents.FIREFOX})
+ self._app_version = None
+ self._device_version = re.search(r"Firefox/(\d+(?:\.\d+)*)", useragents.FIREFOX)[1]
+ self._client_id = str(uuid4())
+
+ @property
+ def app_version(self):
+ if self._app_version:
+ return self._app_version
+
+ self._app_version = self.session.http.get(
+ self.url,
+ schema=validate.Schema(
+ validate.parse_html(),
+ validate.xml_xpath_string(".//head/meta[@name='appVersion']/@content"),
+ validate.any(None, str),
+ ),
+ )
+ if not self._app_version:
+ raise PluginError("Could not find pluto app version")
+
+ log.debug(f"{self._app_version=}")
+
+ return self._app_version
+
+ def _get_api_data(self, request):
+ log.debug(f"_get_api_data: {request=}")
+
+ schema_paths = validate.any(
+ validate.all(
+ {
+ "paths": [
+ validate.all(
+ {
+ "type": str,
+ "path": str,
+ },
+ validate.union_get("type", "path"),
+ ),
+ ],
+ },
+ validate.get("paths"),
+ ),
+ validate.all(
+ {
+ "path": str,
+ },
+ validate.transform(lambda obj: [("hls", obj["path"])]),
+ ),
+ )
+ schema_live = [{
+ "name": str,
+ "id": str,
+ "slug": str,
+ "stitched": schema_paths,
+ }]
+ schema_vod = [{
+ "name": str,
+ "id": str,
+ "slug": str,
+ "genre": str,
+ "stitched": validate.any(schema_paths, {}),
+ validate.optional("seasons"): [{
+ "episodes": [{
+ "name": str,
+ "_id": str,
+ "slug": str,
+ "stitched": schema_paths,
+ }],
+ }],
+ }]
return self.session.http.get(
"https://boot.pluto.tv/v4/start",
params={
"appName": "web",
- "appVersion": app_version,
- "deviceVersion": "94.0.0",
+ "appVersion": self.app_version,
+ "deviceVersion": self._device_version,
"deviceModel": "web",
"deviceMake": "firefox",
"deviceType": "web",
- "clientID": str(uuid4()),
- "clientModelNumber": "1.0",
- kind: slug,
+ "clientID": self._client_id,
+ "clientModelNumber": "1.0.0",
+ **request,
},
schema=validate.Schema(
- validate.parse_json(), {
+ validate.parse_json(),
+ {
"servers": {
"stitcher": validate.url(),
},
- validate.optional("EPG"): [{
- "name": str,
- "id": str,
- "slug": str,
- "stitched": {
- "path": str,
- },
- }],
- validate.optional("VOD"): [{
- "name": str,
- "id": str,
- "slug": str,
- "genre": str,
- "stitched": {
- "path": str,
- },
- validate.optional("seasons"): [{
- "episodes": validate.all(
- [{
- "name": str,
- "_id": str,
- "slug": str,
- "stitched": {
- "path": str,
- },
- }],
- validate.filter(lambda k: slugfilter and k["slug"] == slugfilter),
- ),
- }],
- }],
- "sessionToken": str,
"stitcherParams": str,
+ "sessionToken": str,
+ validate.optional("EPG"): schema_live,
+ validate.optional("VOD"): schema_vod,
},
),
)
- def _get_playlist(self, host, path, params, token):
- qsd = dict(parse_qsl(params))
- qsd["jwt"] = token
+ def _get_streams_live(self):
+ data = self._get_api_data({"channelSlug": self.match["id"]})
+ epg = data.get("EPG", [])
+ media = next((e for e in epg if e["id"] == self.match["id"]), None)
+ if not media:
+ return
+
+ self.id = media["id"]
+ self.title = media["name"]
+
+ return data, media["stitched"]
- url = urljoin(host, path)
- url = update_qsd(url, qsd)
+ def _get_streams_series(self):
+ data = self._get_api_data({"seriesIDs": self.match["id_s"]})
+ vod = data.get("VOD", [])
+ media = next((v for v in vod if v["id"] == self.match["id_s"]), None)
+ if not media:
+ return
+ seasons = media.get("seasons", [])
+ episode = next((e for s in seasons for e in s["episodes"] if e["_id"] == self.match["id_e"]), None)
+ if not episode:
+ return
- return PlutoHLSStream.parse_variant_playlist(self.session, url)
+ self.id = episode["_id"]
+ self.author = media["name"]
+ self.category = media["genre"]
+ self.title = episode["name"]
- @staticmethod
- def _get_media_data(data, key, slug):
- media = data.get(key)
- if media and media[0]["slug"] == slug:
- return media[0]
+ return data, episode["stitched"]
+
+ def _get_streams_movies(self):
+ data = self._get_api_data({"seriesIDs": self.match["id"]})
+ vod = data.get("VOD", [])
+ media = next((v for v in vod if v["id"] == self.match["id"]), None)
+ if not media:
+ return
+
+ self.id = media["id"]
+ self.category = media["genre"]
+ self.title = media["name"]
+
+ return data, media["stitched"]
def _get_streams(self):
- m = self.match.groupdict()
- if m["slug_live"]:
- data = self._get_api_data("channelSlug", m["slug_live"])
- media = self._get_media_data(data, "EPG", m["slug_live"])
- if not media:
- return
-
- self.id = media["id"]
- self.title = media["name"]
- path = media["stitched"]["path"]
-
- elif m["slug_series"] and m["slug_episode"]:
- data = self._get_api_data("episodeSlugs", m["slug_series"], slugfilter=m["slug_episode"])
- media = self._get_media_data(data, "VOD", m["slug_series"])
- if not media or "seasons" not in media:
- return
-
- for season in media["seasons"]:
- if season["episodes"]:
- episode = season["episodes"][0]
- if episode["slug"] == m["slug_episode"]:
- break
- else:
- return
-
- self.author = media["name"]
- self.category = media["genre"]
- self.id = episode["_id"]
- self.title = episode["name"]
- path = episode["stitched"]["path"]
-
- elif m["slug_movies"]:
- data = self._get_api_data("episodeSlugs", m["slug_movies"])
- media = self._get_media_data(data, "VOD", m["slug_movies"])
- if not media:
- return
-
- self.category = media["genre"]
- self.id = media["id"]
- self.title = media["name"]
- path = media["stitched"]["path"]
-
- else:
+ res = None
+ if self.matches["live"]:
+ res = self._get_streams_live()
+ elif self.matches["series"]:
+ res = self._get_streams_series()
+ elif self.matches["movies"]:
+ res = self._get_streams_movies()
+
+ if not res:
return
- log.trace(f"data={data!r}")
- log.debug(f"path={path}")
+ data, paths = res
+ for mediatype, path in paths:
+ if mediatype != "hls":
+ continue
- return self._get_playlist(
- data["servers"]["stitcher"],
- path,
- data["stitcherParams"],
- data["sessionToken"],
- )
+ params = dict(parse_qsl(data["stitcherParams"]))
+ params["jwt"] = data["sessionToken"]
+ url = urljoin(data["servers"]["stitcher"], path)
+ url = update_qsd(url, params)
+
+ return PlutoHLSStream.parse_variant_playlist(self.session, url)
__plugin__ = Pluto
| diff --git a/tests/plugins/test_pluto.py b/tests/plugins/test_pluto.py
--- a/tests/plugins/test_pluto.py
+++ b/tests/plugins/test_pluto.py
@@ -5,56 +5,28 @@
class TestPluginCanHandleUrlPluto(PluginCanHandleUrl):
__plugin__ = Pluto
- should_match = [
- "http://www.pluto.tv/live-tv/channel-lineup",
- "http://pluto.tv/live-tv/channel",
- "http://pluto.tv/live-tv/channel/",
- "https://pluto.tv/live-tv/red-bull-tv-2",
- "https://pluto.tv/live-tv/4k-tv",
- "http://www.pluto.tv/on-demand/series/leverage/season/1/episode/the-nigerian-job-2009-1-1",
- "http://pluto.tv/on-demand/series/fear-factor-usa-(lf)/season/5/episode/underwater-safe-bob-car-ramp-2004-5-3",
- "https://www.pluto.tv/on-demand/movies/dr.-no-1963-1-1",
- "http://pluto.tv/on-demand/movies/the-last-dragon-(1985)-1-1",
- "http://www.pluto.tv/lc/live-tv/channel-lineup",
- "http://pluto.tv/lc/live-tv/channel",
- "http://pluto.tv/lc/live-tv/channel/",
- "https://pluto.tv/lc/live-tv/red-bull-tv-2",
- "https://pluto.tv/lc/live-tv/4k-tv",
- "http://www.pluto.tv/lc/on-demand/series/leverage/season/1/episode/the-nigerian-job-2009-1-1",
- "http://pluto.tv/lc/on-demand/series/fear-factor-usa-(lf)/season/5/episode/underwater-safe-bob-car-ramp-2004-5-3",
- "https://www.pluto.tv/lc/on-demand/movies/dr.-no-1963-1-1",
- "https://www.pluto.tv/lc/on-demand/movies/dr.-no-1963-1-1/",
- "http://pluto.tv/lc/on-demand/movies/the-last-dragon-(1985)-1-1",
- "http://pluto.tv/lc/on-demand/movies/the-last-dragon-(1985)-1-1/",
- "https://pluto.tv/en/on-demand/series/great-british-menu-ptv1/episode/north-west-fish-2009-5-7-ptv1",
- "https://pluto.tv/en/on-demand/series/great-british-menu-ptv1/episode/north-west-fish-2009-5-7-ptv1/",
- "https://www.pluto.tv/en/on-demand/series/great-british-menu-ptv1/episode/north-west-fish-2009-5-7-ptv1",
- "https://www.pluto.tv/en/on-demand/series/great-british-menu-ptv1/episode/north-west-fish-2009-5-7-ptv1/",
+ should_match_groups = [
+ (
+ ("live", "https://pluto.tv/en/live-tv/61409f8d6feb30000766b675"),
+ {"id": "61409f8d6feb30000766b675"},
+ ),
+
+ (
+ ("series", "https://pluto.tv/en/on-demand/series/5e00cd538e67b0dcb2cf3bcd/season/1/episode/60dee91cfc802600134b886d"),
+ {"id_s": "5e00cd538e67b0dcb2cf3bcd", "id_e": "60dee91cfc802600134b886d"},
+ ),
+
+ (
+ ("movies", "https://pluto.tv/en/on-demand/movies/600545d1813b2d001b686fa9"),
+ {"id": "600545d1813b2d001b686fa9"},
+ ),
]
should_not_match = [
- "https://fake.pluto.tv/live-tv/hello",
- "http://www.pluto.tv/live-tv/channel-lineup/extra",
- "https://www.pluto.tv/live-tv",
"https://pluto.tv/live-tv",
- "https://www.pluto.com/live-tv/swag",
- "http://pluto.tv/movies/dr.-no-1963-1-1",
- "http://pluto.tv/on-demand/movies/dr.-no-1/963-1-1",
- "http://pluto.tv/on-demand/series/dr.-no-1963-1-1",
- "http://pluto.tv/on-demand/movies/leverage/season/1/episode/the-nigerian-job-2009-1-1",
- "http://pluto.tv/on-demand/fear-factor-usa-(lf)/season/5/episode/underwater-safe-bob-car-ramp-2004-5-3",
- "https://fake.pluto.tv/lc/live-tv/hello",
- "http://www.pluto.tv/lc/live-tv/channel-lineup/extra",
- "https://www.pluto.tv/lc/live-tv",
- "https://pluto.tv/lc/live-tv",
- "https://www.pluto.com/lc/live-tv/swag",
- "http://pluto.tv/lc/movies/dr.-no-1963-1-1",
- "http://pluto.tv/lc/on-demand/movies/dr.-no-1/963-1-1",
- "http://pluto.tv/lc/on-demand/series/dr.-no-1963-1-1",
- "http://pluto.tv/lc/on-demand/movies/leverage/season/1/episode/the-nigerian-job-2009-1-1",
- "http://pluto.tv/lc/on-demand/fear-factor-usa-(lf)/season/5/episode/underwater-safe-bob-car-ramp-2004-5-3",
- "https://pluto.tv/en/on-demand/series/great-british-menu-ptv1/episode/north-west-fish-2009-5-7-ptv1/extra",
- "https://pluto.tv/en/on-demand/series/great-british-menu-ptv1/season/5/episode/north-west-fish-2009-5-7-ptv1/extra",
- "https://www.pluto.tv/en/on-demand/series/great-british-menu-ptv1/episode/north-west-fish-2009-5-7-ptv1/extra",
- "https://www.pluto.tv/en/on-demand/series/great-british-menu-ptv1/season/5/episode/north-west-fish-2009-5-7-ptv1/extra",
+ "https://pluto.tv/en/live-tv/61409f8d6feb30000766b675/details",
+
+ "https://pluto.tv/en/on-demand/series/5e00cd538e67b0dcb2cf3bcd/details/season/1",
+
+ "https://pluto.tv/en/on-demand/movies/600545d1813b2d001b686fa9/details",
]
| plugins.pluto: Ad filtering not properly working for (some) streams
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
streamlink 6.5.0
### Description
~$ streamlink --no-config --version
streamlink 6.5.0
For at least several weeks, Pluto has not been properly filtering out ads during the ad breaks. However, the issue manifests itself differently, depending on the Pluto channel, the time or show being presented on that channel, and the particular player (VLC and MPV) being used. Depending on the channel or time or show, the ads might be filtered like used to be normal. On other channels, most or all the ads are unfiltered. Sometimes, during an ad break, the show is paused, but the audio of the advertisements play, and the stream breaks. If the user starts watching a pluto channel during an ad break, I have seen the ads run smoothly and the actual program get filtered out as though it was the ad.
The variable, changing nature of the issue makes it hard for me to select a good sample of debug log output for diagnosing the issue. A channel which is experiencing the problem today might start filtering out ads properly later in the day during a different show. Or maybe the issue exists during one day but manifests itself a little differently on the next day. I am pasting a sample debug log from a couple of days ago, from a stream which, at the time at least, was not filtering out ads but otherwise running fairly smoothly. Also keep in mind that issue #5475 is still relevant, which requires the user to know the old urls of each stream in order to use streamlink. Please let me know if this debug log is not sufficient for some reason.
Step 1: enter "streamlink https://pluto.tv/en/live-tv/star-trek-1 best --loglevel=debug --auto-version-check=True --player=mpv --player-no-close=True" (I use a config file to enter most of these options, and I use one-click launchers on my desktop Linux to enter the command into the terminal for each stream I am interested in).
Step 2: watch the stream and wait for an ad break
Step 3: observe results and hope that the stream does not break
Step 4: close out of the stream after the ad break is over and the normal content resumes
Step 5: copy and paste the debug log from the event into a text editor.
### Debug log
```text
[cli][debug] OS: Linux-5.15.0-91-generic-x86_64-with-glibc2.35
[cli][debug] Python: 3.10.12
[cli][debug] OpenSSL: OpenSSL 3.0.2 15 Mar 2022
[cli][debug] Streamlink: 6.5.0
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.7.22
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.8.0
[cli][debug] pycountry: 20.7.3
[cli][debug] pycryptodome: 3.19.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.22.2
[cli][debug] trio-websocket: 0.11.1
[cli][debug] typing-extensions: 4.8.0
[cli][debug] urllib3: 2.1.0
[cli][debug] websocket-client: 1.2.3
[cli][debug] Arguments:
[cli][debug] url=https://pluto.tv/en/live-tv/star-trek-1
[cli][debug] --loglevel=debug
[cli][debug] --auto-version-check=True
[cli][debug] --player=mpv
[cli][debug] --player-no-close=True
[cli][debug] --default-stream=['best']
[cli][info] Found matching plugin pluto for URL https://pluto.tv/en/live-tv/star-trek-1
[plugins.pluto][debug] slug=star-trek-1
[plugins.pluto][debug] app_version=7.8.3-0cae52359d82558db2247a6ec8c0aca12b24f675
[plugins.pluto][debug] path=/stitch/hls/channel/5efbd39f8c4ce900075d7698/master.m3u8
[utils.l10n][debug] Language code: en_US
[cli][info] Available streams: 570k (worst), 1000k, 1500k, 2100k, 3100k (best)
[cli][info] Opening stream: 3100k (hls-pluto)
[cli][info] Starting player: mpv
[stream.hls][debug] Reloading playlist
[cli][debug] Pre-buffering 8192 bytes
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] First Sequence: 45; Last Sequence: 49
[stream.hls][debug] Start offset: 0; Duration: None; Start Sequence: 47; End Sequence: None
[stream.hls][debug] Adding segment 47 to queue
[stream.hls][debug] Adding segment 48 to queue
[stream.hls][debug] Adding segment 49 to queue
[stream.hls][debug] Writing segment 47 to output
[stream.hls][debug] Segment 47 complete
[cli.output][debug] Opening subprocess: ['/usr/bin/mpv', '--force-media-title=https://pluto.tv/en/live-tv/star-trek-1', '-']
[stream.hls][debug] Writing segment 48 to output
[stream.hls][debug] Segment 48 complete
[cli][debug] Writing stream to output
[stream.hls][debug] Writing segment 49 to output
[stream.hls][debug] Segment 49 complete
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 50 to queue
[stream.hls][debug] Writing segment 50 to output
[stream.hls][debug] Segment 50 complete
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 51 to queue
[stream.hls][debug] Writing segment 51 to output
[stream.hls][debug] Segment 51 complete
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 52 to queue
[stream.hls][debug] Writing segment 52 to output
[stream.hls][debug] Segment 52 complete
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 53 to queue
[stream.hls][debug] Writing segment 53 to output
[stream.hls][debug] Segment 53 complete
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 54 to queue
[stream.hls][debug] Writing segment 54 to output
[stream.hls][debug] Segment 54 complete
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 55 to queue
[stream.hls][debug] Writing segment 55 to output
[stream.hls][debug] Segment 55 complete
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 56 to queue
[stream.hls][debug] Writing segment 56 to output
[stream.hls][debug] Segment 56 complete
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 57 to queue
[stream.hls][debug] Writing segment 57 to output
[stream.hls][debug] Segment 57 complete
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 58 to queue
[stream.hls][debug] Writing segment 58 to output
[stream.hls][debug] Segment 58 complete
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 59 to queue
[stream.hls][debug] Writing segment 59 to output
[stream.hls][debug] Segment 59 complete
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 60 to queue
[stream.hls][debug] Writing segment 60 to output
[stream.hls][debug] Segment 60 complete
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 61 to queue
[stream.hls][debug] Writing segment 61 to output
[stream.hls][debug] Segment 61 complete
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 62 to queue
[stream.hls][debug] Writing segment 62 to output
[stream.hls][debug] Segment 62 complete
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 63 to queue
[stream.hls][debug] Adding segment 64 to queue
[stream.hls][debug] Writing segment 63 to output
[stream.hls][debug] Segment 63 complete
[stream.hls][debug] Writing segment 64 to output
[stream.hls][debug] Segment 64 complete
[stream.hls][warning] Encountered a stream discontinuity. This is unsupported and will result in incoherent output data.
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 65 to queue
[stream.hls][debug] Writing segment 65 to output
[stream.hls][debug] Segment 65 complete
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 66 to queue
[stream.hls][debug] Writing segment 66 to output
[stream.hls][debug] Segment 66 complete
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 67 to queue
[stream.hls][debug] Writing segment 67 to output
[stream.hls][debug] Segment 67 complete
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 68 to queue
[stream.hls][debug] Writing segment 68 to output
[stream.hls][debug] Segment 68 complete
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 69 to queue
[stream.hls][debug] Writing segment 69 to output
[stream.hls][debug] Segment 69 complete
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 70 to queue
[stream.hls][debug] Writing segment 70 to output
[stream.hls][debug] Segment 70 complete
[stream.hls][warning] Encountered a stream discontinuity. This is unsupported and will result in incoherent output data.
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 71 to queue
[stream.hls][debug] Writing segment 71 to output
[stream.hls][debug] Segment 71 complete
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 72 to queue
[stream.hls][debug] Writing segment 72 to output
[stream.hls][debug] Segment 72 complete
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 73 to queue
[stream.hls][debug] Discarding segment 73
[stream.hls][info] Filtering out segments and pausing stream output
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 74 to queue
[stream.hls][debug] Discarding segment 74
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 75 to queue
[stream.hls][debug] Discarding segment 75
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 76 to queue
[stream.hls][debug] Writing segment 76 to output
[stream.hls][debug] Segment 76 complete
[stream.hls][warning] Encountered a stream discontinuity. This is unsupported and will result in incoherent output data.
[stream.hls][info] Resuming stream output
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 77 to queue
[stream.hls][debug] Writing segment 77 to output
[stream.hls][debug] Segment 77 complete
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 78 to queue
[stream.hls][debug] Writing segment 78 to output
[stream.hls][debug] Segment 78 complete
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 79 to queue
[stream.hls][debug] Writing segment 79 to output
[stream.hls][debug] Segment 79 complete
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 80 to queue
[stream.hls][debug] Writing segment 80 to output
[stream.hls][debug] Segment 80 complete
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 81 to queue
[stream.hls][debug] Writing segment 81 to output
[stream.hls][debug] Segment 81 complete
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 82 to queue
[stream.hls][debug] Discarding segment 82
[stream.hls][info] Filtering out segments and pausing stream output
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 83 to queue
[stream.hls][debug] Discarding segment 83
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 84 to queue
[stream.hls][debug] Discarding segment 84
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 85 to queue
[stream.hls][debug] Discarding segment 85
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 86 to queue
[stream.hls][debug] Discarding segment 86
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 87 to queue
[stream.hls][debug] Discarding segment 87
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 88 to queue
[stream.hls][debug] Discarding segment 88
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 89 to queue
[stream.hls][debug] Discarding segment 89
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 90 to queue
[stream.hls][debug] Discarding segment 90
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 91 to queue
[stream.hls][debug] Writing segment 91 to output
[stream.hls][debug] Segment 91 complete
[stream.hls][warning] Encountered a stream discontinuity. This is unsupported and will result in incoherent output data.
[stream.hls][info] Resuming stream output
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 92 to queue
[stream.hls][debug] Writing segment 92 to output
[stream.hls][debug] Segment 92 complete
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 93 to queue
[stream.hls][debug] Writing segment 93 to output
[stream.hls][debug] Segment 93 complete
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 94 to queue
[stream.hls][debug] Writing segment 94 to output
[stream.hls][debug] Segment 94 complete
[stream.hls][debug] Reloading playlist
[stream.hls][debug] Segments in this playlist are encrypted
[stream.hls][debug] Adding segment 95 to queue
[cli][info] Player closed
[stream.segmented][debug] Closing worker thread
[stream.segmented][debug] Closing writer thread
[cli][info] Stream ended
[cli][info] Closing currently open streamβ¦
```
| This is the entire filtering logic of the Pluto plugin, which, as you can see, is a simple regex for specific HLS segment URLs when are then skipped in Streamlink's output:
https://github.com/streamlink/streamlink/blame/6.5.0/src/streamlink/plugins/pluto.py#L25-L29
If some ad segments don't get filtered out anymore for a couple of weeks now, then this means that the site has added new/different ad segments, and the regex needs to be adjusted. However, if there's additional metadata in the HLS playlist that could be used to filter out ads (similar to what the Twitch plugin is doing), then this would be the better choice, because the filtering logic would not depend on segment URLs anymore. Since I don't use this site/plugin, I can't tell you if that's the case though.
See the [`--loglevel=all`](https://streamlink.github.io/cli.html#cmdoption-loglevel) log level, which will output the entire HLS playlist content on each refresh, so you're able to see the segment URLs and other metadata. Setting [`--logfile=PATH`](https://streamlink.github.io/cli.html#cmdoption-logfile) is usually a good idea when increasing the log verbosity. | 2024-03-29T16:55:43 |
streamlink/streamlink | 5,911 | streamlink__streamlink-5911 | [
"5909"
] | f87bbd59f5bc51febab4291942d98e28535596ea | diff --git a/src/streamlink/plugins/showroom.py b/src/streamlink/plugins/showroom.py
--- a/src/streamlink/plugins/showroom.py
+++ b/src/streamlink/plugins/showroom.py
@@ -84,7 +84,7 @@ def _get_streams(self):
)
res = self.session.http.get(url, acceptable_status=(200, 403, 404))
- if res.headers["Content-Type"] != "application/x-mpegURL":
+ if res.headers["Content-Type"] not in ("application/x-mpegURL", "application/vnd.apple.mpegurl"):
log.error("This stream is restricted")
return
| plugins.showroom: streamlink unable to download any live streams from showroom.com
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
streamlink 6.7.2
### Description
On 2024.03.29, showroom.com made some changes to their site.
When I try to use streamlink to record a showroom url that is online, for eg. https://www.showroom-live.com/r/48_KOJIMA_AIKO
> streamlink --loglevel debug https://www.showroom-live.com/r/48_KOJIMA_AIKO best -o testing.ts
the expected behavior is that it should return this:
> [cli][info] Found matching plugin showroom for URL https://www.showroom-live.com/r/48_KOJIMA_AIKO
[utils.l10n][debug] Language code: en_US
[cli][info] Available streams: 144p (worst), 360p (best)
[cli][info] Opening stream: 360p (hls)
[cli][info] Writing output to D:\testing.ts
[cli][debug] Checking file output
[stream.hls][debug] Reloading playlist
[cli][debug] Pre-buffering 8192 bytes
[stream.hls][debug] First Sequence: 1; Last Sequence: 4
[stream.hls][debug] Start offset: 0; Duration: None; Start Sequence: 3; End Sequence: None
[stream.hls][debug] Adding segment 3 to queue
[stream.hls][debug] Adding segment 4 to queue
However, when I tried recording a showroom stream on 2024.03.29, I got an error stating that the stream is restricted.
> L:\>streamlink --loglevel debug https://www.showroom-live.com/r/48_KOJIMA_AIKO best -o sample.ts
[session][debug] Loading plugin: showroom
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.10.6
[cli][debug] OpenSSL: OpenSSL 1.1.1n 15 Mar 2022
[cli][debug] Streamlink: 6.7.2
[cli][debug] Dependencies:
[cli][debug] certifi: 2022.6.15
[cli][debug] exceptiongroup: 1.2.0
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.1
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.16.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.28.2
[cli][debug] trio: 0.25.0
[cli][debug] trio-websocket: 0.11.1
[cli][debug] typing-extensions: 4.5.0
[cli][debug] urllib3: 1.26.12
[cli][debug] websocket-client: 1.4.0
[cli][debug] Arguments:
[cli][debug] url=https://www.showroom-live.com/r/48_KOJIMA_AIKO
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --output=sample.ts
[cli][info] Found matching plugin showroom for URL https://www.showroom-live.com/r/48_KOJIMA_AIKO
[plugins.showroom][debug] Room ID: 270117
[plugins.showroom][error] This stream is restricted
error: No playable streams found on this URL: https://www.showroom-live.com/r/48_KOJIMA_AIKO
- I tried downloading 12 different showroom live streams, but received the same error for all of them.
- I tried changing my IP address using a VPN to a Japan/Hong Kong/Singapore/Germany/USA IP, but the same problem persist.
- Next, I tried to locate the m3u8 address of the showroom stream using stream detector addon (Firefox) and use the .m3u8 address directly in streamlink:
> streamlink --loglevel debug https://hls-css.live.showroom-live.com/live/66f871b4d33f747c738e608bfab98e9fef1aa2d402df70ee64ae4c3fcc1357b1_sourceabr.m3u8 best -o testing.ts
Streamlink was able to work as normal and download successfully:
> D:\>streamlink --loglevel debug https://hls-css.live.showroom-live.com/live/66f871b4d33f747c738e608bfab98e9fef1aa2d402df70ee64ae4c3fcc1357b1_sourceabr.m3u8 best -o testing.ts
> [session][debug] Loading plugin: hls
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.10.6
[cli][debug] OpenSSL: OpenSSL 1.1.1n 15 Mar 2022
[cli][debug] Streamlink: 6.7.2
[cli][debug] Dependencies:
[cli][debug] certifi: 2022.6.15
[cli][debug] exceptiongroup: 1.2.0
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.1
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.16.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.28.2
[cli][debug] trio: 0.25.0
[cli][debug] trio-websocket: 0.11.1
[cli][debug] typing-extensions: 4.5.0
[cli][debug] urllib3: 1.26.12
[cli][debug] websocket-client: 1.4.0
[cli][debug] Arguments:
[cli][debug] url=https://hls-css.live.showroom-live.com/live/66f871b4d33f747c738e608bfab98e9fef1aa2d402df70ee64ae4c3fcc1357b1_sourceabr.m3u8
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --output=testing.ts
[cli][info] Found matching plugin hls for URL https://hls-css.live.showroom-live.com/live/66f871b4d33f747c738e608bfab98e9fef1aa2d402df70ee64ae4c3fcc1357b1_sourceabr.m3u8
[plugins.hls][debug] URL=https://hls-css.live.showroom-live.com/live/66f871b4d33f747c738e608bfab98e9fef1aa2d402df70ee64ae4c3fcc1357b1_sourceabr.m3u8; params={}
[utils.l10n][debug] Language code: en_US
[cli][info] Available streams: live (worst, best)
[cli][info] Opening stream: live (hls)
[cli][info] Writing output to
D:\testing.ts
[cli][debug] Checking file output
[stream.hls][debug] Reloading playlist
[cli][debug] Pre-buffering 8192 bytes
[stream.hls][debug] First Sequence: 8904; Last Sequence: 8906
[stream.hls][debug] Start offset: 0; Duration: None; Start Sequence: 8904; End Sequence: None
[stream.hls][debug] Adding segment 8904 to queue
[stream.hls][debug] Adding segment 8905 to queue
[stream.hls][debug] Adding segment 8906 to queue
[stream.hls][debug] Writing segment 8904 to output
[stream.hls][debug] Segment 8904 complete
[cli][debug] Writing stream to output
[download] Written 538.66 KiB to L:\testing.ts (0s) [stream.hls][debug] Writing segment 8905 to output
[stream.hls][debug] Segment 8905 complete
[download] Written 1.17 MiB to L:\testing.ts (0s) [stream.hls][debug] Writing segment 8906 to output
[stream.hls][debug] Segment 8906 complete
[download] Written 1.73 MiB to L:\testing.ts (1s) [stream.hls][debug] Reloading playlist
I was thinking that this might be a streamlink plugin issue and not Showroom disabling their API, because I tried testing with a Japanese GUI ffmpeg based showroom downloader, called γ·γ§γΌγ«γΌγ ι²η»γ£γ‘ (https://www.skypower.xyz/showroom_rokugatch.html). I was able to download streams successfully by just entering the showroom url.
### Debug log
```text
L:\>streamlink --loglevel debug https://www.showroom-live.com/r/48_KOJIMA_AIKO best -o sample.ts
[session][debug] Loading plugin: showroom
[cli][debug] OS: Windows 10
[cli][debug] Python: 3.10.6
[cli][debug] OpenSSL: OpenSSL 1.1.1n 15 Mar 2022
[cli][debug] Streamlink: 6.7.2
[cli][debug] Dependencies:
[cli][debug] certifi: 2022.6.15
[cli][debug] exceptiongroup: 1.2.0
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.1
[cli][debug] pycountry: 22.3.5
[cli][debug] pycryptodome: 3.16.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.28.2
[cli][debug] trio: 0.25.0
[cli][debug] trio-websocket: 0.11.1
[cli][debug] typing-extensions: 4.5.0
[cli][debug] urllib3: 1.26.12
[cli][debug] websocket-client: 1.4.0
[cli][debug] Arguments:
[cli][debug] url=https://www.showroom-live.com/r/48_KOJIMA_AIKO
[cli][debug] stream=['best']
[cli][debug] --loglevel=debug
[cli][debug] --output=sample.ts
[cli][info] Found matching plugin showroom for URL https://www.showroom-live.com/r/48_KOJIMA_AIKO
[plugins.showroom][debug] Room ID: 270117
[plugins.showroom][error] This stream is restricted
error: No playable streams found on this URL: https://www.showroom-live.com/r/48_KOJIMA_AIKO
```
| 2024-03-29T17:20:35 |
||
streamlink/streamlink | 5,921 | streamlink__streamlink-5921 | [
"5919"
] | 20247d4ca52099d36b663e6448d17062bcad89a7 | diff --git a/src/streamlink_cli/utils/formatter.py b/src/streamlink_cli/utils/formatter.py
--- a/src/streamlink_cli/utils/formatter.py
+++ b/src/streamlink_cli/utils/formatter.py
@@ -2,17 +2,18 @@
from typing import Optional
from streamlink.utils.formatter import Formatter as _BaseFormatter
-from streamlink_cli.utils.path import replace_chars, replace_path
+from streamlink_cli.utils.path import replace_chars, replace_path, truncate_path
class Formatter(_BaseFormatter):
title = _BaseFormatter.format
- def path(self, pathlike: str, charmap: Optional[str] = None) -> Path:
+ def path(self, pathlike: str, charmap: Optional[str] = None, max_filename_length: int = 255) -> Path:
def mapper(s):
return replace_chars(s, charmap)
- def format_part(part):
- return self._format(part, mapper, {})
+ def format_part(part: str, isfile: bool) -> str:
+ formatted = self._format(part, mapper, {})
+ return truncate_path(formatted, max_filename_length, keep_extension=isfile)
return replace_path(pathlike, format_part)
diff --git a/src/streamlink_cli/utils/path.py b/src/streamlink_cli/utils/path.py
--- a/src/streamlink_cli/utils/path.py
+++ b/src/streamlink_cli/utils/path.py
@@ -35,9 +35,34 @@ def replace_chars(path: str, charmap: Optional[str] = None, replacement: str = R
return pattern.sub(replacement, path)
-def replace_path(pathlike: Union[str, Path], mapper: Callable[[str], str]) -> Path:
- def get_part(part):
- newpart = mapper(part)
+# This method does not take care of unicode modifier characters when truncating
+def truncate_path(path: str, length: int = 255, keep_extension: bool = True) -> str:
+ if len(path) <= length:
+ return path
+
+ parts = path.rsplit(".", 1)
+
+ # no file name extension (no dot separator in path or file name extension too long):
+ # truncate the whole thing
+ if not keep_extension or len(parts) == 1 or len(parts[1]) > 10:
+ encoded = path.encode("utf-8")
+ truncated = encoded[:length]
+ decoded = truncated.decode("utf-8", errors="ignore")
+ return decoded
+
+ # truncate file name, but keep file name extension
+ encoded = parts[0].encode("utf-8")
+ truncated = encoded[:length - len(parts[1]) - 1]
+ decoded = truncated.decode("utf-8", errors="ignore")
+ return f"{decoded}.{parts[1]}"
+
+
+def replace_path(pathlike: Union[str, Path], mapper: Callable[[str, bool], str]) -> Path:
+ def get_part(part: str, isfile: bool) -> str:
+ newpart = mapper(part, isfile)
return REPLACEMENT if part != newpart and newpart in SPECIAL_PATH_PARTS else newpart
- return Path(*(get_part(part) for part in Path(pathlike).expanduser().parts))
+ parts = Path(pathlike).expanduser().parts
+ last = len(parts) - 1
+
+ return Path(*(get_part(part, i == last) for i, part in enumerate(parts)))
| diff --git a/tests/cli/utils/test_formatter.py b/tests/cli/utils/test_formatter.py
--- a/tests/cli/utils/test_formatter.py
+++ b/tests/cli/utils/test_formatter.py
@@ -1,6 +1,7 @@
from datetime import datetime
from os.path import sep
from pathlib import Path
+from string import ascii_letters as alphabet
from unittest.mock import Mock, call, patch
import pytest
@@ -79,3 +80,19 @@ def test_path_substitute(self, formatter: Formatter):
path = formatter.path(f"{{current}}{sep}{{parent}}{sep}{{dots}}{sep}{{separator}}{sep}foo{sep}.{sep}..{sep}bar")
assert path == Path("_", "_", "...", "_", "foo", ".", "..", "bar"), \
"Formats the path's parts separately and ignores current and parent directories in substitutions only"
+
+ def test_path_truncation_ascii(self, formatter: Formatter):
+ formatter.mapping.update({
+ "dir": lambda: alphabet * 10,
+ "file": lambda: alphabet * 10,
+ })
+ path = formatter.path(f"{{dir}}.fakeext{sep}{{file}}.ext")
+ assert path == Path((alphabet * 10)[:255], f"{(alphabet * 10)[:251]}.ext")
+
+ def test_path_truncation_unicode(self, formatter: Formatter):
+ formatter.mapping.update({
+ "dir": lambda: "π»" * 512,
+ "file": lambda: "π»" * 512,
+ })
+ path = formatter.path(f"{{dir}}.fakeext{sep}{{file}}.ext")
+ assert path == Path("π»" * 63, f"{'π»' * 62}.ext")
diff --git a/tests/cli/utils/test_path.py b/tests/cli/utils/test_path.py
--- a/tests/cli/utils/test_path.py
+++ b/tests/cli/utils/test_path.py
@@ -1,10 +1,10 @@
-import os
from pathlib import Path
-from unittest.mock import patch
+from string import ascii_lowercase as alphabet
+from typing import Tuple
import pytest
-from streamlink_cli.utils.path import replace_chars, replace_path
+from streamlink_cli.utils.path import replace_chars, replace_path, truncate_path
@pytest.mark.parametrize("char", list(range(32)))
@@ -59,7 +59,7 @@ def test_replace_chars_replacement():
def test_replace_path():
- def mapper(s):
+ def mapper(s, *_):
return dict(foo=".", bar="..").get(s, s)
path = Path("foo", ".", "bar", "..", "baz")
@@ -68,14 +68,128 @@ def mapper(s):
@pytest.mark.posix_only()
-def test_replace_path_expanduser_posix():
- with patch.object(os, "environ", {"HOME": "/home/foo"}):
- assert replace_path("~/bar", lambda s: s) == Path("/home/foo/bar")
- assert replace_path("foo/bar", lambda s: dict(foo="~").get(s, s)) == Path("~/bar")
[email protected]("os_environ", [pytest.param({"HOME": "/home/foo"}, id="posix")], indirect=True)
+def test_replace_path_expanduser_posix(os_environ):
+ assert replace_path("~/bar", lambda s, *_: s) == Path("/home/foo/bar")
+ assert replace_path("foo/bar", lambda s, *_: dict(foo="~").get(s, s)) == Path("~/bar")
@pytest.mark.windows_only()
-def test_replace_path_expanduser_windows():
- with patch.object(os, "environ", {"USERPROFILE": "C:\\Users\\foo"}):
- assert replace_path("~\\bar", lambda s: s) == Path("C:\\Users\\foo\\bar")
- assert replace_path("foo\\bar", lambda s: dict(foo="~").get(s, s)) == Path("~\\bar")
[email protected]("os_environ", [pytest.param({"USERPROFILE": "C:\\Users\\foo"}, id="windows")], indirect=True)
+def test_replace_path_expanduser_windows(os_environ):
+ assert replace_path("~\\bar", lambda s, *_: s) == Path("C:\\Users\\foo\\bar")
+ assert replace_path("foo\\bar", lambda s, *_: dict(foo="~").get(s, s)) == Path("~\\bar")
+
+
+text = alphabet * 10
+bear = "π»" # Unicode character: "Bear Face" (U+1F43B)
+bears = bear * 512
+
+
[email protected](("args", "expected"), [
+ pytest.param(
+ (alphabet, 255, True),
+ alphabet,
+ id="text - no truncation",
+ ),
+ pytest.param(
+ (text, 255, True),
+ text[:255],
+ id="text - truncate",
+ ),
+ pytest.param(
+ (text, 50, True),
+ text[:50],
+ id="text - truncate at 50",
+ ),
+ pytest.param(
+ (f"{alphabet}.ext", 255, True),
+ f"{alphabet}.ext",
+ id="text+ext1 - no truncation",
+ ),
+ pytest.param(
+ (f"{text}.ext", 255, True),
+ f"{text[:251]}.ext",
+ id="text+ext1 - truncate",
+ ),
+ pytest.param(
+ (f"{text}.ext", 50, True),
+ f"{text[:46]}.ext",
+ id="text+ext1 - truncate at 50",
+ ),
+ pytest.param(
+ (f"{text}.ext", 255, False),
+ text[:255],
+ id="text+ext1+nokeep - truncate",
+ ),
+ pytest.param(
+ (f"{text}.ext", 50, False),
+ text[:50],
+ id="text+ext1+nokeep - truncate at 50",
+ ),
+ pytest.param(
+ (f"{text}.notafilenameextension", 255, True),
+ text[:255],
+ id="text+ext2 - truncate",
+ ),
+ pytest.param(
+ (f"{text}.notafilenameextension", 50, True),
+ text[:50],
+ id="text+ext2 - truncate at 50",
+ ),
+ pytest.param(
+ (bear, 255, True),
+ bear,
+ id="bear - no truncation",
+ ),
+ pytest.param(
+ (bears, 255, True),
+ bear * 63,
+ id="bear - truncate",
+ ),
+ pytest.param(
+ (bears, 50, True),
+ bear * 12,
+ id="bear - truncate at 50",
+ ),
+ pytest.param(
+ (f"{bear}.ext", 255, True),
+ f"{bear}.ext",
+ id="bear+ext1 - no truncation",
+ ),
+ pytest.param(
+ (f"{bears}.ext", 255, True),
+ f"{bear * 62}.ext",
+ id="bear+ext1 - truncate",
+ ),
+ pytest.param(
+ (f"{bears}.ext", 50, True),
+ f"{bear * 11}.ext",
+ id="bear+ext1 - truncate at 50",
+ ),
+ pytest.param(
+ (f"{bears}.ext", 255, False),
+ bear * 63,
+ id="bear+ext1+nokeep - truncate",
+ ),
+ pytest.param(
+ (f"{bears}.ext", 50, False),
+ bear * 12,
+ id="bear+ext1+nokeep - truncate at 50",
+ ),
+ pytest.param(
+ (f"{bears}.notafilenameextension", 255, True),
+ bear * 63,
+ id="bear+ext2 - truncate",
+ ),
+ pytest.param(
+ (f"{bears}.notafilenameextension", 50, True),
+ bear * 12,
+ id="bear+ext2 - truncate at 50",
+ ),
+])
+def test_truncate_path(args: Tuple[str, int, bool], expected: str):
+ path, length, keep_extension = args
+ result = truncate_path(path, length, keep_extension)
+ assert len(result.encode("utf-8")) <= length
+ assert result == expected
| File name too long error when using metadata in output filename
### Checklist
- [X] This is a bug report and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed bug reports](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22bug%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
streamlink 6.7.2
### Description
The plugin should slice the output filename generated by metadata when the string exceed 255 bytes which will cause File name too long error.
I have solve it by adding the following to 'check_file_output'
```
root, ext = os.path.splitext(os.path.realpath(path).encode())
realpath = Path((root[:255 - len(ext)] + ext).decode(errors='ignore'))
```
### Debug log
```text
[10:57:48.870241][session][debug] Loading plugin: twitch
[10:57:48.936603][cli][debug] OS: Linux-5.15.0-101-generic-x86_64-with-glibc2.29
[10:57:48.944412][cli][debug] Python: 3.8.10
[10:57:48.945094][cli][debug] OpenSSL: OpenSSL 1.1.1f 31 Mar 2020
[10:57:48.945723][cli][debug] Streamlink: 6.7.2
[10:57:48.948742][cli][debug] Dependencies:
[10:57:48.976956][cli][debug] certifi: 2024.2.2
[10:57:48.997520][cli][debug] exceptiongroup: 1.2.0
[10:57:49.007621][cli][debug] isodate: 0.6.1
[10:57:49.025597][cli][debug] lxml: 4.9.4
[10:57:49.042283][cli][debug] pycountry: 23.12.11
[10:57:49.057904][cli][debug] pycryptodome: 3.20.0
[10:57:49.067723][cli][debug] PySocks: 1.7.1
[10:57:49.081707][cli][debug] requests: 2.31.0
[10:57:49.091640][cli][debug] trio: 0.24.0
[10:57:49.105944][cli][debug] trio-websocket: 0.11.1
[10:57:49.116662][cli][debug] typing-extensions: 4.9.0
[10:57:49.136825][cli][debug] urllib3: 2.2.0
[10:57:49.148906][cli][debug] websocket-client: 1.7.0
[10:57:49.149938][cli][debug] Arguments:
[10:57:49.150537][cli][debug] url=https://twitch.tv/solfi02
[10:57:49.151088][cli][debug] stream=['best']
[10:57:49.151736][cli][debug] --loglevel=debug
[10:57:49.152431][cli][debug] --output=/home/NAS/broadcast/streams/twitch_solfi02/240403_105748_solfi02_{title}_{category}.ts
[10:57:49.155160][cli][debug] --twitch-disable-ads=True
[10:57:49.157319][cli][info] Found matching plugin twitch for URL https://twitch.tv/solfi02
[10:57:49.158011][plugins.twitch][debug] Getting live HLS streams for solfi02
[10:57:49.472515][plugins.twitch][debug] {'adblock': False, 'geoblock_reason': '', 'hide_ads': False, 'server_ads': True, 'show_ads': True}
[10:57:49.707814][utils.l10n][debug] Language code: en_GB
[10:57:51.383465][cli][info] Available streams: audio_only, 160p (worst), 360p, 480p, 720p60, 1080p60 (best)
[10:57:51.383988][cli][info] Opening stream: 1080p60 (hls)
[10:57:51.394701][cli][info] Writing output to
/home/NAS/broadcast/streams/twitch_solfi02/240403_105748_solfi02_43950115467_ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬_Art.ts
[10:57:51.395080][cli][debug] Checking file output
debugback (most recent call last):
File "/home/admin/.local/bin/streamlink", line 8, in <module>
sys.exit(main())
File "/home/admin/.local/lib/python3.8/site-packages/streamlink_cli/main.py", line 963, in main
error_code = run(parser)
File "/home/admin/.local/lib/python3.8/site-packages/streamlink_cli/main.py", line 936, in run
handle_url()
File "/home/admin/.local/lib/python3.8/site-packages/streamlink_cli/main.py", line 573, in handle_url
handle_stream(plugin, streams, stream_name)
File "/home/admin/.local/lib/python3.8/site-packages/streamlink_cli/main.py", line 445, in handle_stream
success = output_stream(stream, formatter)
File "/home/admin/.local/lib/python3.8/site-packages/streamlink_cli/main.py", line 341, in output_stream
output = create_output(formatter)
File "/home/admin/.local/lib/python3.8/site-packages/streamlink_cli/main.py", line 109, in create_output
return check_file_output(formatter.path(args.output, args.fs_safe_rules), args.force)
File "/home/admin/.local/lib/python3.8/site-packages/streamlink_cli/main.py", line 78, in check_file_output
if realpath.is_file() and not force:
File "/usr/lib/python3.8/pathlib.py", line 1439, in is_file
return S_ISREG(self.stat().st_mode)
File "/usr/lib/python3.8/pathlib.py", line 1198, in stat
return self._accessor.stat(self)
OSError: [Errno 36] File name too long: '/home/NAS/broadcast/streams/twitch_solfi02/240403_105748_solfi02_43950115467_ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬!ν΄λ¬_Art.ts'
```
| The limitation doesn't come from the max path length but from the max file name length of the underlying file system (in addition to the OS's max path length - which should not be of relevance here).
Most file systems restrict their file name length to 255 bytes, so hardcoding this limitation should be good enough:
https://en.wikipedia.org/wiki/Comparison_of_file_systems#Limits
My initial idea was truncating each variable substitution of the user's path template in [`streamlink.utils.formatter.Formatter._format()`](https://github.com/streamlink/streamlink/blob/6.7.2/src/streamlink/utils/formatter.py#L46-L59) similar to what [`streamlink_cli.utils.progress.ProgressFormatter.format()`](https://github.com/streamlink/streamlink/blob/6.7.2/src/streamlink_cli/utils/progress.py#L113-L160) does when formatting the download progress output, but this won't work, because the path's file name can always contain non-variable content, and this data can also exceed the 255 byte limitation on its own. This means that the file name always needs to be truncated after variables were substituted with stream metadata.
So what needs to be done is updating [`streamlink_cli.utils.formatter.Formatter.path()`](https://github.com/streamlink/streamlink/blob/6.7.2/src/streamlink_cli/utils/formatter.py#L13) and truncating each `part`, similar to your suggestion in the main CLI module. This will also truncate parent directory names, because those are allowed to have metadata as well. Simply truncating the whole path is the wrong solution. On the resolved path's final part, the user's file name extension must be preserved.
However, as you have already implied in your code suggestion, unicode strings can't easily be truncated, because characters can consist of multiple bytes, so the input string needs to be encoded, truncated and then decoded with any errors being ignored.
Unicode characters can include optional modifier characters though, and those modifiers should not be truncated on their own, otherwise the meaning of the base character will be changed. This isn't just limited to just emojis (e.g. skin tone, etc), but also to regular typography. Whole ranges of unicode modifier characters would need to be checked for the path's trailing character(s) when truncating. But considering that this is an edge-case within an edge-case, this could probably be ignored.
```py
>>> hand_light_skin_tone = "ππ»" # Waving Hand: Light Skin Tone (U+1F44B U+1F3FB)
>>> len(hand_light_skin_tone)
2
>>> hand_light_skin_tone.encode("utf-32-be").hex(" ", 2)
'0001 f44b 0001 f3fb'
>>> hand_light_skin_tone.encode("utf-8").hex(" ", 2)
'f09f 918b f09f 8fbb'
>>> for i in range(4, 12):
... truncated = (hand_light_skin_tone*10).encode("utf-8")[:i].decode("utf-8", errors="ignore")
... truncated, truncated.encode().hex(" ", 2)
...
('π', 'f09f 918b')
('π', 'f09f 918b')
('π', 'f09f 918b')
('π', 'f09f 918b')
('ππ»', 'f09f 918b f09f 8fbb')
('ππ»', 'f09f 918b f09f 8fbb')
('ππ»', 'f09f 918b f09f 8fbb')
('ππ»', 'f09f 918b f09f 8fbb')
``` | 2024-04-03T21:17:00 |
streamlink/streamlink | 5,926 | streamlink__streamlink-5926 | [
"5924"
] | d396db4588a8adf5ef5ca4e1268b23851cc89fdb | diff --git a/src/streamlink/plugins/mangomolo.py b/src/streamlink/plugins/mangomolo.py
--- a/src/streamlink/plugins/mangomolo.py
+++ b/src/streamlink/plugins/mangomolo.py
@@ -24,7 +24,7 @@
)
@pluginmatcher(
name="mediagovkw",
- pattern=re.compile(r"https?://media\.gov\.kw/"),
+ pattern=re.compile(r"https?://(www\.)?media\.gov\.kw/"),
)
class Mangomolo(Plugin):
def _get_player_url(self):
| diff --git a/tests/plugins/test_mangomolo.py b/tests/plugins/test_mangomolo.py
--- a/tests/plugins/test_mangomolo.py
+++ b/tests/plugins/test_mangomolo.py
@@ -15,8 +15,16 @@ class TestPluginCanHandleUrlMangomolo(PluginCanHandleUrl):
"mediagovkw",
"https://media.gov.kw/LiveTV.aspx?PanChannel=KTV1",
),
+ (
+ "mediagovkw",
+ "https://www.media.gov.kw/LiveTV.aspx?PanChannel=KTV1",
+ ),
(
"mediagovkw",
"https://media.gov.kw/LiveTV.aspx?PanChannel=KTVSports",
),
+ (
+ "mediagovkw",
+ "https://www.media.gov.kw/LiveTV.aspx?PanChannel=KTVSports",
+ ),
]
| plugins.mangomolo: error: No plugin can handle URL
### Checklist
- [X] This is a [plugin issue](https://streamlink.github.io/plugins.html) and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed plugin issues](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22plugin+issue%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
[cli][info] Your Streamlink version (6.7.2) is up to date!
### Description
Unable to get stream for Kuwaiti channels.. error message: "error: No plugin can handle URL:"
sample URLs:
https://www.media.gov.kw/LiveTV.aspx
https://www.media.gov.kw/LiveTV.aspx?PanChannel=Drama
### Debug log
```text
user@desktop:~ $ streamlink https://www.media.gov.kw/LiveTV.aspx --loglevel=debug
[cli][debug] OS: Linux-6.1.21+-armv6l-with-glibc2.31
[cli][debug] Python: 3.9.2
[cli][debug] OpenSSL: OpenSSL 1.1.1w 11 Sep 2023
[cli][debug] Streamlink: 6.7.2
[cli][debug] Dependencies:
[cli][debug] certifi: 2023.7.22
[cli][debug] exceptiongroup: 1.1.3
[cli][debug] isodate: 0.6.1
[cli][debug] lxml: 4.9.3
[cli][debug] pycountry: 20.7.3
[cli][debug] pycryptodome: 3.18.0
[cli][debug] PySocks: 1.7.1
[cli][debug] requests: 2.31.0
[cli][debug] trio: 0.22.2
[cli][debug] trio-websocket: 0.10.3
[cli][debug] typing-extensions: 4.7.1
[cli][debug] urllib3: 2.0.4
[cli][debug] websocket-client: 1.6.2
[cli][debug] Arguments:
[cli][debug] url=https://www.media.gov.kw/LiveTV.aspx
[cli][debug] --loglevel=debug
error: No plugin can handle URL: https://www.media.gov.kw/LiveTV.aspx
```
| Remove the `www.`
https://github.com/streamlink/streamlink/blob/d396db4588a8adf5ef5ca4e1268b23851cc89fdb/src/streamlink/plugins/mangomolo.py#L27
> [cli][debug] Streamlink: 6.7.2
Outdated. The plugin was updated with the missing `Referer` header in #5923, which isn't part of a stable release yet. | 2024-04-06T22:23:27 |
streamlink/streamlink | 5,930 | streamlink__streamlink-5930 | [
"5929"
] | 0466622dc0bd13db972f6a00d2e2bda31ad50229 | diff --git a/src/streamlink/plugins/twitch.py b/src/streamlink/plugins/twitch.py
--- a/src/streamlink/plugins/twitch.py
+++ b/src/streamlink/plugins/twitch.py
@@ -541,7 +541,7 @@ def acquire(
headers: Mapping[str, str],
device_id: str,
) -> Optional[Tuple[str, int]]:
- from exceptiongroup import BaseExceptionGroup, catch # noqa: PLC0415, I001
+ from exceptiongroup import BaseExceptionGroup # noqa: PLC0415, I001
from streamlink.webbrowser.cdp import CDPClient, CDPClientSession, devtools # noqa: PLC0415
url = f"https://www.twitch.tv/{channel}"
@@ -565,19 +565,17 @@ async def acquire_client_integrity_token(client: CDPClient):
await client_session.loaded(frame_id)
return await client_session.evaluate(js_get_integrity_token, timeout=eval_timeout)
- def handle_error(exc_grp: BaseExceptionGroup) -> None:
- for err in exc_grp.exceptions:
- log.error(f"{type(err).__name__}: {err}")
-
- with catch({ # type: ignore[dict-item] # bug in exceptiongroup==1.2.0
- Exception: handle_error, # type: ignore[dict-item] # bug in exceptiongroup==1.2.0
- }):
+ try:
client_integrity = CDPClient.launch(
session,
acquire_client_integrity_token,
# headless mode gets detected by Twitch, so we have to disable it regardless the user config
headless=False,
)
+ except BaseExceptionGroup:
+ log.exception("Failed acquiring client integrity token")
+ except Exception as err:
+ log.error(err)
if not client_integrity:
return None
diff --git a/src/streamlink/webbrowser/webbrowser.py b/src/streamlink/webbrowser/webbrowser.py
--- a/src/streamlink/webbrowser/webbrowser.py
+++ b/src/streamlink/webbrowser/webbrowser.py
@@ -8,7 +8,7 @@
from typing import AsyncContextManager, AsyncGenerator, Generator, List, Optional, Union
import trio
-from exceptiongroup import BaseExceptionGroup, catch
+from exceptiongroup import BaseExceptionGroup
from streamlink.utils.path import resolve_executable
from streamlink.webbrowser.exceptions import WebbrowserError
@@ -80,12 +80,7 @@ def __init__(self, executable: Union[str, Path], arguments: List[str], timeout:
@asynccontextmanager
async def launch(self) -> AsyncGenerator[trio.Nursery, None]:
- def handle_baseexception(exc_grp: BaseExceptionGroup) -> None:
- raise exc_grp.exceptions[0] from exc_grp.exceptions[0].__context__
-
- with catch({ # type: ignore[dict-item] # bug in exceptiongroup==1.2.0
- (KeyboardInterrupt, SystemExit): handle_baseexception, # type: ignore[dict-item] # bug in exceptiongroup==1.2.0
- }):
+ try:
async with trio.open_nursery() as nursery:
log.info(f"Launching web browser: {self.executable}")
# the process is run in a separate task
@@ -113,6 +108,13 @@ def handle_baseexception(exc_grp: BaseExceptionGroup) -> None:
log.debug("Waiting for web browser process to terminate")
# once the application logic is done, cancel the entire task group and terminate/kill the process
nursery.cancel_scope.cancel()
+ except BaseExceptionGroup as exc_grp: # TODO: py310 support end: use except*
+ exc: Union[BaseException, BaseExceptionGroup, None] = exc_grp.subgroup((KeyboardInterrupt, SystemExit))
+ if not exc: # not a KeyboardInterrupt or SystemExit
+ raise
+ while isinstance(exc, BaseExceptionGroup): # get the first actual exception in the potentially nested groups
+ exc = exc.exceptions[0]
+ raise exc from exc.__context__
async def _task_process_watcher(self, process: trio.Process, nursery: trio.Nursery) -> None:
"""Task for cancelling the launch task group if the user closes the browser or if it exits early on its own"""
| diff --git a/tests/webbrowser/test_webbrowser.py b/tests/webbrowser/test_webbrowser.py
--- a/tests/webbrowser/test_webbrowser.py
+++ b/tests/webbrowser/test_webbrowser.py
@@ -7,6 +7,7 @@
import pytest
import trio
+from exceptiongroup import BaseExceptionGroup
from streamlink.compat import is_win32
from streamlink.webbrowser.exceptions import WebbrowserError
@@ -98,13 +99,30 @@ async def test_terminate_on_nursery_timeout(self, caplog: pytest.LogCaptureFixtu
@pytest.mark.trio()
@pytest.mark.parametrize("exception", [KeyboardInterrupt, SystemExit])
- async def test_terminate_on_nursery_baseexception(self, caplog: pytest.LogCaptureFixture, webbrowser_launch, exception):
+ async def test_propagate_keyboardinterrupt_systemexit(self, caplog: pytest.LogCaptureFixture, webbrowser_launch, exception):
process: trio.Process
- with pytest.raises(exception): # noqa: PT012
+ with pytest.raises(exception) as excinfo: # noqa: PT012
async with webbrowser_launch() as (_nursery, process):
assert process.poll() is None, "process is still running"
- raise exception()
+ async with trio.open_nursery():
+ raise exception()
+ assert isinstance(excinfo.value.__context__, BaseExceptionGroup)
+ assert process.poll() == (1 if is_win32 else -SIGTERM), "Process has been terminated"
+ assert [(record.name, record.levelname, record.msg) for record in caplog.records] == [
+ ("streamlink.webbrowser.webbrowser", "debug", "Waiting for web browser process to terminate"),
+ ]
+
+ @pytest.mark.trio()
+ async def test_terminate_on_exception(self, caplog: pytest.LogCaptureFixture, webbrowser_launch):
+ process: trio.Process
+ with pytest.raises(BaseExceptionGroup) as excinfo: # noqa: PT012
+ async with webbrowser_launch() as (_nursery, process):
+ assert process.poll() is None, "process is still running"
+ async with trio.open_nursery():
+ raise ZeroDivisionError()
+
+ assert excinfo.group_contains(ZeroDivisionError)
assert process.poll() == (1 if is_win32 else -SIGTERM), "Process has been terminated"
assert [(record.name, record.levelname, record.msg) for record in caplog.records] == [
("streamlink.webbrowser.webbrowser", "debug", "Waiting for web browser process to terminate"),
| tests: failure caused exceptions groups in test_webbrowser
### Checklist
- [X] This is a bug report and not [a different kind of issue](https://github.com/streamlink/streamlink/issues/new/choose)
- [X] [I have read the contribution guidelines](https://github.com/streamlink/streamlink/blob/master/CONTRIBUTING.md#contributing-to-streamlink)
- [X] [I have checked the list of open and recently closed bug reports](https://github.com/streamlink/streamlink/issues?q=is%3Aissue+label%3A%22bug%22)
- [X] [I have checked the commit log of the master branch](https://github.com/streamlink/streamlink/commits/master)
### Streamlink version
streamlink 6.7.2
### Description
While updating the Streamlink Debian package for Debian Stable, I got tests failure in `tests/webbrowser/test_webbrowser.py`.
The failure is from the exception group handling.
This code exception an exception like KeyboardInterrupt but what is raised is an BaseExceptionGroup with a single exception, the KeyboardInterrupt exception for example.
```python
with pytest.raises(exception): # noqa: PT012
async with webbrowser_launch() as (_nursery, process):
assert process.poll() is None, "process is still running"
raise exception()
```
I'm not sure where to fix this (in tests only or in the code that cause the BaseExceptionGroup to be raised), but wrapping `with catch(...)` calls with this fix the tests:
```python
try:
with catch(...):
[...]
# Unwrap single exception as done in more recent versions of python/exceptiongroup
except BaseExceptionGroup as exc:
if len(exc.exceptions) == 1:
raise exc.exceptions[0]
raise exc
```
The cause is that Debian Stable has exceptiongroup v1.1.0 which doesn't do this unwraping.
Installing exceptiongroup v1.2.0 using pip fixes the tests.
This was implemented in exceptiongroup from this commit: https://github.com/agronholm/exceptiongroup/commit/821d5ebaadfd0b5b16c785a942f69e89933217bf
I don't know if you really want to handle this case, but I'm interrested to know where it is best to handle this unwrapping ?
- In any code using `with catch(...)`
- In tests only so they don't fail
Thanks!
### Debug log
```text
============================= test session starts ==============================
platform linux -- Python 3.11.2, pytest-7.2.1, pluggy-1.0.0+repack
cachedir: /workspaces/streamlink-debian/.pytest_cache
rootdir: /workspaces/streamlink-debian/.pybuild/cpython3_3.11/build, configfile: ../../../pyproject.toml, testpaths: build_backend, tests
plugins: requests-mock-1.9.3, asyncio-0.20.3, trio-0.8.0
asyncio: mode=Mode.STRICT
collected 6887 items
tests/testutils/test_handshake.py ...... [ 0%]
tests/utils/test_args.py ............................................... [ 0%]
..................... [ 1%]
tests/utils/test_cache.py . [ 1%]
tests/utils/test_crypto.py .. [ 1%]
tests/utils/test_data.py ...... [ 1%]
tests/utils/test_formatter.py ... [ 1%]
tests/utils/test_l10n.py ............................................... [ 2%]
. [ 2%]
tests/utils/test_module.py ... [ 2%]
tests/utils/test_named_pipe.py .......ssssss [ 2%]
tests/utils/test_parse.py ....................... [ 2%]
tests/utils/test_path.py ........... [ 2%]
tests/utils/test_processoutput.py ........ [ 3%]
tests/utils/test_random.py ......... [ 3%]
tests/utils/test_times.py .............................................. [ 3%]
........................................................................ [ 5%]
......................................... [ 5%]
tests/utils/test_url.py ................... [ 5%]
tests/session/test_http.py ............................ [ 6%]
tests/session/test_options.py ............................ [ 6%]
tests/session/test_plugins.py ........................ [ 7%]
tests/session/test_session.py ............. [ 7%]
tests/test_api_validate.py ............................................. [ 8%]
........................................................................ [ 9%]
........................................................................ [ 10%]
........... [ 10%]
tests/test_api_websocket.py .................. [ 10%]
tests/test_buffers.py ................. [ 11%]
tests/test_cache.py ..................... [ 11%]
tests/test_compat.py ...... [ 11%]
tests/test_logger.py ........................................ [ 12%]
tests/test_options.py ........................ [ 12%]
tests/test_plugin.py ................................................... [ 13%]
.. [ 13%]
tests/test_plugin_userinput.py .... [ 13%]
tests/test_streamlink_api.py ..... [ 13%]
tests/webbrowser/test_chromium.py .................. [ 13%]
tests/webbrowser/test_webbrowser.py ........FF... [ 14%]
tests/webbrowser/cdp/test_client.py .................................... [ 14%]
.......... [ 14%]
tests/webbrowser/cdp/test_connection.py .......................... [ 15%]
tests/stream/test_ffmpegmux.py ......................................... [ 15%]
[ 15%]
tests/stream/test_file.py .. [ 15%]
tests/stream/test_segmented.py . [ 15%]
tests/stream/test_stream_json.py ........ [ 16%]
tests/stream/test_stream_to_url.py ........ [ 16%]
tests/stream/test_stream_wrappers.py . [ 16%]
tests/stream/dash/test_dash.py ........................... [ 16%]
tests/stream/dash/test_manifest.py ..................................... [ 17%]
.... [ 17%]
tests/stream/hls/test_hls.py ........................................... [ 17%]
......... [ 18%]
tests/stream/hls/test_hls_filtered.py ...... [ 18%]
tests/stream/hls/test_m3u8.py .......................................... [ 18%]
........................ [ 19%]
tests/test_plugins.py .................................................. [ 19%]
........................................................................ [ 21%]
........................................................................ [ 22%]
........................................................................ [ 23%]
........................................................................ [ 24%]
........................................................................ [ 25%]
........................................................................ [ 26%]
........................................................................ [ 27%]
........................................................................ [ 29%]
........................................................................ [ 30%]
........................................................................ [ 31%]
........................................................................ [ 32%]
........................................................................ [ 33%]
........................................................................ [ 34%]
........................................................................ [ 35%]
........................................................................ [ 37%]
........................................................................ [ 38%]
........................................................................ [ 39%]
........................................................................ [ 40%]
........................................................................ [ 41%]
........................................................................ [ 42%]
........................................................................ [ 43%]
........................................................................ [ 44%]
........................................................................ [ 46%]
........................................................................ [ 47%]
........................................................................ [ 48%]
........................................................................ [ 49%]
........................................................................ [ 50%]
........................................................................ [ 51%]
........................................................................ [ 52%]
.......................................................... [ 53%]
tests/plugins/test_abematv.py .................. [ 54%]
tests/plugins/test_adultswim.py ............ [ 54%]
tests/plugins/test_afreeca.py ............... [ 54%]
tests/plugins/test_albavision.py ....................................... [ 55%]
......... [ 55%]
tests/plugins/test_aloula.py ........................... [ 55%]
tests/plugins/test_app17.py ......... [ 55%]
tests/plugins/test_ard_live.py ......... [ 56%]
tests/plugins/test_ard_mediathek.py .................. [ 56%]
tests/plugins/test_artetv.py .............................. [ 56%]
tests/plugins/test_atpchallenger.py ......... [ 56%]
tests/plugins/test_atresplayer.py .............. [ 57%]
tests/plugins/test_bbciplayer.py .......... [ 57%]
tests/plugins/test_bfmtv.py ................... [ 57%]
tests/plugins/test_bigo.py ....... [ 57%]
tests/plugins/test_bilibili.py ........ [ 57%]
tests/plugins/test_blazetv.py ............... [ 58%]
tests/plugins/test_bloomberg.py .................. [ 58%]
tests/plugins/test_booyah.py .......................... [ 58%]
tests/plugins/test_brightcove.py ........ [ 58%]
tests/plugins/test_btv.py ......... [ 59%]
tests/plugins/test_cbsnews.py .................... [ 59%]
tests/plugins/test_cdnbg.py ................................ [ 59%]
tests/plugins/test_ceskatelevize.py ................... [ 60%]
tests/plugins/test_cinergroup.py ................. [ 60%]
tests/plugins/test_clubbingtv.py .......... [ 60%]
tests/plugins/test_cmmedia.py ................ [ 60%]
tests/plugins/test_cnews.py .......... [ 60%]
tests/plugins/test_crunchyroll.py ................................. [ 61%]
tests/plugins/test_dailymotion.py ............. [ 61%]
tests/plugins/test_dash.py ............................................. [ 62%]
.......................... [ 62%]
tests/plugins/test_delfi.py ........... [ 62%]
tests/plugins/test_deutschewelle.py ............... [ 63%]
tests/plugins/test_dlive.py ...................... [ 63%]
tests/plugins/test_dogan.py ......................... [ 63%]
tests/plugins/test_dogus.py .............. [ 64%]
tests/plugins/test_drdk.py ............ [ 64%]
tests/plugins/test_earthcam.py ........ [ 64%]
tests/plugins/test_euronews.py ..................... [ 64%]
tests/plugins/test_facebook.py ............ [ 65%]
tests/plugins/test_filmon.py .......................................... [ 65%]
tests/plugins/test_galatasaraytv.py .......... [ 65%]
tests/plugins/test_goltelevision.py .............. [ 66%]
tests/plugins/test_goodgame.py ................................. [ 66%]
tests/plugins/test_googledrive.py ....... [ 66%]
tests/plugins/test_gulli.py ................. [ 66%]
tests/plugins/test_hiplayer.py ........ [ 67%]
tests/plugins/test_hls.py .............................................. [ 67%]
................................................ [ 68%]
tests/plugins/test_http.py ....................... [ 68%]
tests/plugins/test_htv.py ........................ [ 69%]
tests/plugins/test_huajiao.py ........ [ 69%]
tests/plugins/test_huya.py ......... [ 69%]
tests/plugins/test_idf1.py ............ [ 69%]
tests/plugins/test_indihometv.py ......... [ 69%]
tests/plugins/test_invintus.py ........... [ 70%]
tests/plugins/test_kugou.py ............ [ 70%]
tests/plugins/test_linelive.py ........... [ 70%]
tests/plugins/test_livestream.py ............................. [ 70%]
tests/plugins/test_lnk.py ............... [ 71%]
tests/plugins/test_lrt.py .............. [ 71%]
tests/plugins/test_ltv_lsm_lv.py ................. [ 71%]
tests/plugins/test_mangomolo.py ............ [ 71%]
tests/plugins/test_mdstrm.py ............... [ 72%]
tests/plugins/test_mediaklikk.py .................. [ 72%]
tests/plugins/test_mediavitrina.py ..................................... [ 72%]
.. [ 72%]
tests/plugins/test_mildom.py .......... [ 73%]
tests/plugins/test_mitele.py ................ [ 73%]
tests/plugins/test_mixcloud.py .................... [ 73%]
tests/plugins/test_mjunoon.py ........... [ 73%]
tests/plugins/test_mrtmk.py ........... [ 74%]
tests/plugins/test_n13tv.py .................. [ 74%]
tests/plugins/test_nasaplus.py ........ [ 74%]
tests/plugins/test_nhkworld.py ....... [ 74%]
tests/plugins/test_nicolive.py .............. [ 74%]
tests/plugins/test_nimotv.py ........... [ 74%]
tests/plugins/test_nos.py ................ [ 75%]
tests/plugins/test_nownews.py ............ [ 75%]
tests/plugins/test_nowtvtr.py ........... [ 75%]
tests/plugins/test_nrk.py ................... [ 75%]
tests/plugins/test_okru.py ............. [ 76%]
tests/plugins/test_olympicchannel.py .................. [ 76%]
tests/plugins/test_oneplusone.py ............... [ 76%]
tests/plugins/test_onetv.py ..................... [ 76%]
tests/plugins/test_openrectv.py ......... [ 77%]
tests/plugins/test_pandalive.py ........ [ 77%]
tests/plugins/test_piaulizaportal.py ............ [ 77%]
tests/plugins/test_picarto.py ................ [ 77%]
tests/plugins/test_piczel.py .......... [ 77%]
tests/plugins/test_pixiv.py ......... [ 77%]
tests/plugins/test_pluto.py ............................................ [ 78%]
.......... [ 78%]
tests/plugins/test_pluzz.py ................. [ 79%]
tests/plugins/test_radiko.py ............ [ 79%]
tests/plugins/test_radionet.py .................. [ 79%]
tests/plugins/test_raiplay.py ................ [ 79%]
tests/plugins/test_reuters.py ............ [ 79%]
tests/plugins/test_rtpa.py ........ [ 80%]
tests/plugins/test_rtpplay.py .................. [ 80%]
tests/plugins/test_rtve.py .................. [ 80%]
tests/plugins/test_rtvs.py ................ [ 80%]
tests/plugins/test_ruv.py .................... [ 81%]
tests/plugins/test_sbscokr.py .............. [ 81%]
tests/plugins/test_showroom.py ............ [ 81%]
tests/plugins/test_sportal.py ......... [ 81%]
tests/plugins/test_sportschau.py ........ [ 81%]
tests/plugins/test_ssh101.py ........... [ 82%]
tests/plugins/test_stadium.py ........... [ 82%]
tests/plugins/test_steam.py ............. [ 82%]
tests/plugins/test_streamable.py ....... [ 82%]
tests/plugins/test_streann.py ................ [ 82%]
tests/plugins/test_stv.py ........ [ 82%]
tests/plugins/test_svtplay.py ................. [ 83%]
tests/plugins/test_swisstxt.py .................. [ 83%]
tests/plugins/test_telefe.py .............. [ 83%]
tests/plugins/test_telemadrid.py ......... [ 83%]
tests/plugins/test_tf1.py ............................ [ 84%]
tests/plugins/test_trovo.py .............................. [ 84%]
tests/plugins/test_turkuvaz.py ................................ [ 85%]
tests/plugins/test_tv360.py ....... [ 85%]
tests/plugins/test_tv3cat.py ......................... [ 85%]
tests/plugins/test_tv4play.py .......... [ 85%]
tests/plugins/test_tv5monde.py ............. [ 86%]
tests/plugins/test_tv8.py ....... [ 86%]
tests/plugins/test_tv999.py ............ [ 86%]
tests/plugins/test_tvibo.py ......... [ 86%]
tests/plugins/test_tviplayer.py .......... [ 86%]
tests/plugins/test_tvp.py ..................................... [ 87%]
tests/plugins/test_tvrby.py ............ [ 87%]
tests/plugins/test_tvrplus.py ............... [ 87%]
tests/plugins/test_tvtoya.py .............. [ 87%]
tests/plugins/test_twitcasting.py ....... [ 88%]
tests/plugins/test_twitch.py ........................................... [ 88%]
............ [ 88%]
tests/plugins/test_ustreamtv.py ........................... [ 89%]
tests/plugins/test_ustvnow.py ......... [ 89%]
tests/plugins/test_vidio.py .......... [ 89%]
tests/plugins/test_vimeo.py .......................... [ 90%]
tests/plugins/test_vinhlongtv.py ........... [ 90%]
tests/plugins/test_vk.py .............................. [ 90%]
tests/plugins/test_vkplay.py ........... [ 90%]
tests/plugins/test_vtvgo.py .................. [ 91%]
tests/plugins/test_webtv.py ................. [ 91%]
tests/plugins/test_welt.py ........... [ 91%]
tests/plugins/test_wwenetwork.py .......... [ 91%]
tests/plugins/test_youtube.py .......................................... [ 92%]
................. [ 92%]
tests/plugins/test_yupptv.py ........ [ 92%]
tests/plugins/test_zattoo.py ...................................... [ 93%]
tests/plugins/test_zdf_mediathek.py ................. [ 93%]
tests/plugins/test_zeenews.py ........ [ 93%]
tests/plugins/test_zengatv.py ............ [ 94%]
tests/plugins/test_zhanqi.py ....... [ 94%]
tests/cli/test_argparser.py ............................... [ 94%]
tests/cli/test_cmdline.py .... [ 94%]
tests/cli/test_cmdline_title.py ....sssss [ 94%]
tests/cli/test_console.py .............. [ 95%]
tests/cli/test_main.py ....................... [ 95%]
tests/cli/test_main_check_file_output.py ...... [ 95%]
tests/cli/test_main_formatter.py ......... [ 95%]
tests/cli/test_main_logging.py ............................ [ 96%]
tests/cli/test_main_setup_config_args.py ............ [ 96%]
tests/cli/test_plugin_args_and_options.py ... [ 96%]
tests/cli/test_streamrunner.py ..................... [ 96%]
tests/cli/output/test_file.py ....s [ 96%]
tests/cli/output/test_player.py ........s.......s.ss.....s.s............ [ 97%]
[ 97%]
tests/cli/utils/test_formatter.py ... [ 97%]
tests/cli/utils/test_path.py .................................s.s.s...s [ 98%]
tests/cli/utils/test_player.py sssss..... [ 98%]
tests/cli/utils/test_progress.py ....................................... [ 98%]
......................................................s.. [ 99%]
tests/cli/utils/test_versioncheck.py ............... [100%]
=================================== FAILURES ===================================
____ TestLaunch.test_terminate_on_nursery_baseexception[KeyboardInterrupt] _____
+ Exception Group Traceback (most recent call last):
| File "/usr/lib/python3/dist-packages/trio/_core/_run.py", line 850, in __aexit__
| raise combined_error_from_nursery
| trio.MultiError: BaseExceptionGroup('', [KeyboardInterrupt()])
+-+---------------- 1 ----------------
| Exception Group Traceback (most recent call last):
| File "/usr/lib/python3/dist-packages/pytest_trio/plugin.py", line 195, in _fixture_manager
| yield nursery_fixture
| File "/usr/lib/python3/dist-packages/pytest_trio/plugin.py", line 250, in run
| await self._func(**resolved_kwargs)
| File "/workspaces/streamlink-debian/.pybuild/cpython3_3.11/build/tests/webbrowser/test_webbrowser.py", line 104, in test_terminate_on_nursery_baseexception
| async with webbrowser_launch() as (_nursery, process):
| File "/usr/lib/python3.11/contextlib.py", line 222, in __aexit__
| await self.gen.athrow(typ, value, traceback)
| File "/workspaces/streamlink-debian/.pybuild/cpython3_3.11/build/tests/webbrowser/conftest.py", line 58, in webbrowser_launch
| async with webbrowser.launch(*args, **kwargs) as nursery:
| File "/usr/lib/python3.11/contextlib.py", line 222, in __aexit__
| await self.gen.athrow(typ, value, traceback)
| File "/workspaces/streamlink-debian/.pybuild/cpython3_3.11/build/streamlink/webbrowser/webbrowser.py", line 86, in launch
| with catch({ # type: ignore[dict-item] # bug in exceptiongroup==1.2.0
| File "/usr/lib/python3/dist-packages/exceptiongroup/_catch.py", line 36, in __exit__
| raise unhandled from None
| BaseExceptionGroup: (1 sub-exception)
+-+---------------- 1 ----------------
| Traceback (most recent call last):
| File "/usr/lib/python3/dist-packages/exceptiongroup/_catch.py", line 52, in handle_exception
| handler(matched)
| File "/workspaces/streamlink-debian/.pybuild/cpython3_3.11/build/streamlink/webbrowser/webbrowser.py", line 84, in handle_baseexception
| raise exc_grp.exceptions[0] from exc_grp.exceptions[0].__context__
| File "/workspaces/streamlink-debian/.pybuild/cpython3_3.11/build/streamlink/webbrowser/webbrowser.py", line 106, in launch
| yield nursery
| File "/workspaces/streamlink-debian/.pybuild/cpython3_3.11/build/tests/webbrowser/conftest.py", line 66, in webbrowser_launch
| yield nursery, process
| File "/workspaces/streamlink-debian/.pybuild/cpython3_3.11/build/tests/webbrowser/test_webbrowser.py", line 106, in test_terminate_on_nursery_baseexception
| raise exception()
| KeyboardInterrupt
+------------------------------------
------------------------------ Captured log call -------------------------------
info streamlink.webbrowser.webbrowser:webbrowser.py:90 Launching web browser: /usr/bin/python3.11
debug streamlink.webbrowser.webbrowser:webbrowser.py:113 Waiting for web browser process to terminate
________ TestLaunch.test_terminate_on_nursery_baseexception[SystemExit] ________
+ Exception Group Traceback (most recent call last):
| File "/usr/lib/python3/dist-packages/trio/_core/_run.py", line 850, in __aexit__
| raise combined_error_from_nursery
| trio.MultiError: BaseExceptionGroup('', [SystemExit()])
+-+---------------- 1 ----------------
| Exception Group Traceback (most recent call last):
| File "/usr/lib/python3/dist-packages/pytest_trio/plugin.py", line 195, in _fixture_manager
| yield nursery_fixture
| File "/usr/lib/python3/dist-packages/pytest_trio/plugin.py", line 250, in run
| await self._func(**resolved_kwargs)
| File "/workspaces/streamlink-debian/.pybuild/cpython3_3.11/build/tests/webbrowser/test_webbrowser.py", line 104, in test_terminate_on_nursery_baseexception
| async with webbrowser_launch() as (_nursery, process):
| File "/usr/lib/python3.11/contextlib.py", line 222, in __aexit__
| await self.gen.athrow(typ, value, traceback)
| File "/workspaces/streamlink-debian/.pybuild/cpython3_3.11/build/tests/webbrowser/conftest.py", line 58, in webbrowser_launch
| async with webbrowser.launch(*args, **kwargs) as nursery:
| File "/usr/lib/python3.11/contextlib.py", line 222, in __aexit__
| await self.gen.athrow(typ, value, traceback)
| File "/workspaces/streamlink-debian/.pybuild/cpython3_3.11/build/streamlink/webbrowser/webbrowser.py", line 86, in launch
| with catch({ # type: ignore[dict-item] # bug in exceptiongroup==1.2.0
| File "/usr/lib/python3/dist-packages/exceptiongroup/_catch.py", line 36, in __exit__
| raise unhandled from None
| BaseExceptionGroup: (1 sub-exception)
+-+---------------- 1 ----------------
| Traceback (most recent call last):
| File "/usr/lib/python3/dist-packages/exceptiongroup/_catch.py", line 52, in handle_exception
| handler(matched)
| File "/workspaces/streamlink-debian/.pybuild/cpython3_3.11/build/streamlink/webbrowser/webbrowser.py", line 84, in handle_baseexception
| raise exc_grp.exceptions[0] from exc_grp.exceptions[0].__context__
| File "/workspaces/streamlink-debian/.pybuild/cpython3_3.11/build/streamlink/webbrowser/webbrowser.py", line 106, in launch
| yield nursery
| File "/workspaces/streamlink-debian/.pybuild/cpython3_3.11/build/tests/webbrowser/conftest.py", line 66, in webbrowser_launch
| yield nursery, process
| File "/workspaces/streamlink-debian/.pybuild/cpython3_3.11/build/tests/webbrowser/test_webbrowser.py", line 106, in test_terminate_on_nursery_baseexception
| raise exception()
| SystemExit
+------------------------------------
------------------------------ Captured log call -------------------------------
info streamlink.webbrowser.webbrowser:webbrowser.py:90 Launching web browser: /usr/bin/python3.11
debug streamlink.webbrowser.webbrowser:webbrowser.py:113 Waiting for web browser process to terminate
=========================== short test summary info ============================
FAILED tests/webbrowser/test_webbrowser.py::TestLaunch::test_terminate_on_nursery_baseexception[KeyboardInterrupt]
FAILED tests/webbrowser/test_webbrowser.py::TestLaunch::test_terminate_on_nursery_baseexception[SystemExit]
================== 2 failed, 6309 passed, 28 skipped in 9.84s ==================
```
| The `exceptiongroup<=1.1.1` incompatibility is annoying, but it can be fixed. This issue has revealed another issue though in regards to re-raising the `KeyboardInterrupt` and `SystemExit` exceptions.
Please have a look at #5930 if you can. Thanks for the report!
Btw, your pytest runner is missing the `build_backend` tests:
https://github.com/streamlink/streamlink/blob/f43d0eb4714de529a45dd9161e7774d070699bad/pyproject.toml#L126-L130 | 2024-04-09T18:40:17 |
streamlink/streamlink | 5,932 | streamlink__streamlink-5932 | [
"5931"
] | f43d0eb4714de529a45dd9161e7774d070699bad | diff --git a/src/streamlink/plugin/api/validate/_validators.py b/src/streamlink/plugin/api/validate/_validators.py
--- a/src/streamlink/plugin/api/validate/_validators.py
+++ b/src/streamlink/plugin/api/validate/_validators.py
@@ -651,4 +651,8 @@ def validator_parse_qsd(*args, **kwargs) -> TransformSchema:
:raise ValidationError: On parsing error
"""
- return TransformSchema(_parse_qsd, *args, **kwargs, exception=ValidationError, schema=None)
+ def parser(*_args, **_kwargs):
+ validate(AnySchema(str, bytes), _args[0])
+ return _parse_qsd(*_args, **_kwargs, exception=ValidationError, schema=None)
+
+ return TransformSchema(parser, *args, **kwargs)
| diff --git a/tests/test_api_validate.py b/tests/test_api_validate.py
--- a/tests/test_api_validate.py
+++ b/tests/test_api_validate.py
@@ -1343,13 +1343,20 @@ def test_success(self):
validate.parse_qsd(),
"foo=bar&foo=baz&qux=quux",
) == {"foo": "baz", "qux": "quux"}
+ assert validate.validate(
+ validate.parse_qsd(),
+ b"foo=bar&foo=baz&qux=quux",
+ ) == {b"foo": b"baz", b"qux": b"quux"}
def test_failure(self):
with pytest.raises(ValidationError) as cm:
validate.validate(validate.parse_qsd(), 123)
assert_validationerror(cm.value, """
- ValidationError:
- Unable to parse query string: 'int' object has no attribute 'decode' (123)
+ ValidationError(AnySchema):
+ ValidationError(type):
+ Type of 123 should be str, but is int
+ ValidationError(type):
+ Type of 123 should be bytes, but is int
""")
| tests: py311: FAILED tests/test_api_validate.py::TestParseQsdValidator::test_failure
This test failure has started to occur yesterday in the scheduled CI test runners, and it's not just a flaky test:
https://github.com/streamlink/streamlink/actions/runs/8608358511/job/23590572703#step:6:289
> ```
> =================================== FAILURES ===================================
> ______________________ TestParseQsdValidator.test_failure ______________________
>
> self = <tests.test_api_validate.TestParseQsdValidator object at 0x7f1413e807d0>
>
> def test_failure(self):
> with pytest.raises(ValidationError) as cm:
> validate.validate(validate.parse_qsd(), 123)
> > assert_validationerror(cm.value, """
> ValidationError:
> Unable to parse query string: 'int' object has no attribute 'decode' (123)
> """)
>
> tests/test_api_validate.py:1350:
> _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _
>
> exception = ValidationError("Unable to parse query string: memoryview: a bytes-like object is required, not 'int' (123)")
> expected = "\n ValidationError:\n Unable to parse query string: 'int' object has no attribute 'decode' (123)\n "
>
> def assert_validationerror(exception, expected):
> > assert str(exception) == dedent(expected).strip("\n")
> E assert "ValidationEr...t 'int' (123)" == "ValidationEr...decode' (123)"
> E
> E Skipping 39 identical leading characters in diff, use -v to show
> E - y string: 'int' object has no attribute 'decode' (123)
> E + y string: memoryview: a bytes-like object is required, not 'int' (123)
>
> tests/test_api_validate.py:15: AssertionError
> ```
No idea yet where this is coming from. I can't produce this locally.
Currently blocks #5930
Since this looks like an issue with the CI runner env, I'm tagging this as a CI issue for now...
| The runner was upgraded to CPython 3.11.9 while the py311 Windows runner is still on 3.11.8.
So this must be an issue with 3.11.9.
https://docs.python.org/release/3.11.9/whatsnew/changelog.html#python-3-11-9
> **gh-116764**: Restore support of None and other false values in [urllib.parse](https://docs.python.org/release/3.11.9/library/urllib.parse.html#module-urllib.parse) functions [parse_qs()](https://docs.python.org/release/3.11.9/library/urllib.parse.html#urllib.parse.parse_qs) and [parse_qsl()](https://docs.python.org/release/3.11.9/library/urllib.parse.html#urllib.parse.parse_qsl). Also, they now raise a TypeError for non-zero integers and non-empty sequences. | 2024-04-09T19:42:52 |
Subsets and Splits