text
stringlengths 2
1.04M
| meta
dict |
---|---|
'use strict';
angular.module('ontimeApp')
.controller('ProjectDetailController', function ($scope, $rootScope, $stateParams, entity, Project) {
$scope.project = entity;
$scope.load = function (id) {
Project.get({id: id}, function(result) {
$scope.project = result;
});
};
var unsubscribe = $rootScope.$on('ontimeApp:projectUpdate', function(event, result) {
$scope.project = result;
});
$scope.$on('$destroy', unsubscribe);
});
| {
"content_hash": "99e7a804ba81526ecb2012039b9b5eb9",
"timestamp": "",
"source": "github",
"line_count": 16,
"max_line_length": 105,
"avg_line_length": 33.625,
"alnum_prop": 0.5613382899628253,
"repo_name": "sandor-balazs/nosql-java",
"id": "6a1629f05fd9cbecbd39476bfb37793f637c19c6",
"size": "538",
"binary": false,
"copies": "2",
"ref": "refs/heads/master",
"path": "cassandra/src/main/webapp/scripts/app/entities/project/project-detail.controller.js",
"mode": "33188",
"license": "bsd-2-clause",
"language": [
{
"name": "ApacheConf",
"bytes": "96556"
},
{
"name": "CSS",
"bytes": "17016"
},
{
"name": "HTML",
"bytes": "968574"
},
{
"name": "Java",
"bytes": "2187353"
},
{
"name": "JavaScript",
"bytes": "1330402"
},
{
"name": "Scala",
"bytes": "241589"
}
],
"symlink_target": ""
} |
using System;
using System.Collections.Generic;
using System.Linq;
using System.Linq.Expressions;
using System.Reflection;
using System.Text;
using Microsoft.Deployment.WindowsInstaller;
using WixSharp.CommonTasks;
using IO = System.IO;
using System.IO;
using System.Diagnostics;
namespace WixSharp
{
/// <summary>
/// Extends <see cref="T:WixSharp.Project"/> with runtime event-driven behavior ans managed UI (see <see cref="T:WixSharp.ManagedUI"/> and
/// <see cref="T:WixSharp.UI.Forms.ManagedForm"/>).
/// <para>
/// The managed project has three very important events that are raised during deployment at run: Load/BeforeInstall/AfterInstall.
/// </para>
/// <remark>
/// ManagedProject still maintains backward compatibility for the all older features. That is why it is important to distinguish the use cases
/// associated with the project class members dedicated to the same problem domain but resolving the problems in different ways:
/// <para><c>UI support</c></para>
/// <para> project.UI - to be used to define native MSI/WiX UI.</para>
/// <para> project.CustomUI - to be used for minor to customization of native MSI/WiX UI and injection of CLR dialogs. </para>
/// <para> project.ManagedUI - to be used to define managed Embedded UI. It allows full customization of the UI</para>
/// <para> </para>
/// <para><c>Events</c></para>
/// <para> project.WixSourceGenerated</para>
/// <para> project.WixSourceFormated</para>
/// <para> project.WixSourceSaved - to be used at compile time to customize WiX source code (XML) generation.</para>
/// <para> </para>
/// <para> project.Load</para>
/// <para> project.BeforeInstall</para>
/// <para> project.AfterInstall - to be used at runtime (msi execution) to customize deployment behaver.</para>
/// </remark>
/// </summary>
/// <example>The following is an example of a simple setup handling the three setup events at runtime.
/// <code>
/// var project = new ManagedProject("ManagedSetup",
/// new Dir(@"%ProgramFiles%\My Company\My Product",
/// new File(@"..\Files\bin\MyApp.exe")));
///
/// project.GUID = new Guid("6f330b47-2577-43ad-9095-1861ba25889b");
///
/// project.ManagedUI = ManagedUI.Empty;
///
/// project.Load += project_Load;
/// project.BeforeInstall += project_BeforeInstall;
/// project.AfterInstall += project_AfterInstall;
///
/// project.BuildMsi();
/// </code>
/// </example>
public partial class ManagedProject : Project
{
//some materials to consider: http://cpiekarski.com/2012/05/18/wix-custom-action-sequencing/
/// <summary>
/// Initializes a new instance of the <see cref="ManagedProject"/> class.
/// </summary>
public ManagedProject()
{
}
/// <summary>
/// Initializes a new instance of the <see cref="ManagedProject"/> class.
/// </summary>
/// <param name="name">The name of the project. Typically it is the name of the product to be installed.</param>
/// <param name="items">The project installable items (e.g. directories, files, registry keys, Custom Actions).</param>
public ManagedProject(string name, params WixObject[] items)
: base(name, items)
{
}
/// <summary>
/// Gets or sets a value indicating whether the full or reduced custom drawing algorithm should be used for rendering the Features dialog
/// tree view control. The reduced algorithm involves no manual positioning of the visual elements so it handles better custom screen resolutions.
/// However it also leads to the slightly less intuitive tree view item appearance.
/// <para>Reduced custom drawing will render disabled tree view items with the text grayed out.</para>
/// <para>Full custom drawing will render disabled tree view items with the checkbox grayed out.</para>
/// </summary>
/// <value>
/// <c>true</c> (default) if custom drawing should be reduced otherwise, <c>false</c>.
/// </value>
public bool MinimalCustomDrawing
{
get
{
return Properties.Where(x => x.Name == "WixSharpUI_TreeNode_TexOnlyDrawing").FirstOrDefault() != null;
}
set
{
if (value)
{
var prop = Properties.Where(x => x.Name == "WixSharpUI_TreeNode_TexOnlyDrawing").FirstOrDefault();
if (prop != null)
prop.Value = "true";
else
this.AddProperty(new Property("WixSharpUI_TreeNode_TexOnlyDrawing", "true"));
}
else
{
Properties = Properties.Where(x => x.Name != "WixSharpUI_TreeNode_TexOnlyDrawing").ToArray();
}
}
}
/// <summary>
/// Event handler of the ManagedSetup for the MSI runtime events.
/// </summary>
/// <param name="e">The <see cref="SetupEventArgs"/> instance containing the event data.</param>
public delegate void SetupEventHandler(SetupEventArgs e);
/// <summary>
/// Occurs on EmbeddedUI initialized but before the first dialog is displayed. It is only invoked if ManagedUI is set.
/// </summary>
public event SetupEventHandler UIInitialized;
/// <summary>
/// Occurs on EmbeddedUI loaded and ShellView (main window) is displayed but before first dialog is positioned within ShellView.
/// It is only invoked if ManagedUI is set.
/// <para>Note that this event is fired on the loading the UI main window thus it's not a good stage for any decision regarding
/// aborting/continuing the whole setup process. That is UILoaded event will ignore any value set to SetupEventArgs.Result by the user.
/// </para>
/// </summary>
public event SetupEventHandler UILoaded;
/// <summary>
/// Occurs before AppSearch standard action.
/// </summary>
public event SetupEventHandler Load;
/// <summary>
/// Occurs before InstallFiles standard action.
/// </summary>
public event SetupEventHandler BeforeInstall;
/// <summary>
/// Occurs after InstallFiles standard action. The event is fired from the elevated execution context.
/// </summary>
public event SetupEventHandler AfterInstall;
/// <summary>
/// An instance of ManagedUI defining MSI UI dialogs sequence. User should set it if he/she wants native MSI dialogs to be
/// replaced by managed ones.
/// </summary>
public IManagedUI ManagedUI;
bool preprocessed = false;
string thisAsm = typeof(ManagedProject).Assembly.Location;
void Bind<T>(Expression<Func<T>> expression, When when = When.Before, Step step = null, bool elevated = false)
{
var name = Reflect.NameOf(expression);
var handler = expression.Compile()() as Delegate;
const string wixSharpProperties = "WIXSHARP_RUNTIME_DATA";
if (handler != null)
{
foreach (string handlerAsm in handler.GetInvocationList().Select(x => x.Method.DeclaringType.Assembly.Location))
{
if (!this.DefaultRefAssemblies.Contains(handlerAsm))
this.DefaultRefAssemblies.Add(handlerAsm);
}
this.Properties = this.Properties.Add(new Property("WixSharp_{0}_Handlers".FormatInline(name), GetHandlersInfo(handler as MulticastDelegate)));
string dllEntry = "WixSharp_{0}_Action".FormatInline(name);
if (step != null)
{
if (elevated)
this.Actions = this.Actions.Add(new ElevatedManagedAction(new Id(dllEntry), dllEntry, thisAsm, Return.check, when, step, Condition.Create("1"))
{
UsesProperties = "WixSharp_{0}_Handlers,{1},{2}".FormatInline(name, wixSharpProperties, DefaultDeferredProperties),
});
else
this.Actions = this.Actions.Add(new ManagedAction(new Id(dllEntry), dllEntry, thisAsm, Return.check, when, step, Condition.Create("1")));
}
}
}
/// <summary>
/// The default properties mapped for use with the deferred custom actions. See <see cref="ManagedAction.UsesProperties"/> for the details.
/// <para>The default value is "INSTALLDIR,UILevel"</para>
/// </summary>
public string DefaultDeferredProperties = "INSTALLDIR,UILevel,ProductName";
override internal void Preprocess()
{
//Debug.Assert(false);
base.Preprocess();
if (!preprocessed)
{
preprocessed = true;
//It is too late to set prerequisites. Launch conditions are evaluated after UI is popped up.
//this.SetNetFxPrerequisite(Condition.Net35_Installed, "Please install .NET v3.5 first.");
string dllEntry = "WixSharp_InitRuntime_Action";
this.AddAction(new ManagedAction(new Id(dllEntry), dllEntry, thisAsm, Return.check, When.Before, Step.AppSearch, Condition.Always));
if (ManagedUI != null)
{
this.AddProperty(new Property("WixSharp_UI_INSTALLDIR", ManagedUI.InstallDirId ?? "INSTALLDIR"));
ManagedUI.BeforeBuild(this);
InjectDialogs("WixSharp_InstallDialogs", ManagedUI.InstallDialogs);
InjectDialogs("WixSharp_ModifyDialogs", ManagedUI.ModifyDialogs);
this.EmbeddedUI = new EmbeddedAssembly(ManagedUI.GetType().Assembly.Location);
this.DefaultRefAssemblies.Add(ManagedUI.GetType().Assembly.Location);
Bind(() => UIInitialized);
Bind(() => UILoaded);
}
Bind(() => Load, When.Before, Step.AppSearch);
Bind(() => BeforeInstall, When.Before, Step.InstallFiles);
Bind(() => AfterInstall, When.After, Step.InstallFiles, true);
}
}
void InjectDialogs(string name, ManagedDialogs dialogs)
{
if (dialogs.Any())
{
var dialogsInfo = new StringBuilder();
foreach (var item in dialogs)
{
if (!this.DefaultRefAssemblies.Contains(item.Assembly.Location))
this.DefaultRefAssemblies.Add(item.Assembly.Location);
var info = GetDialogInfo(item);
ValidateDialogInfo(info);
dialogsInfo.Append(info + "\n");
}
this.AddProperty(new Property(name, dialogsInfo.ToString().Trim()));
}
}
/// <summary>
/// Reads and returns the dialog types from the string definition. This method is to be used by WixSharp assembly.
/// </summary>
/// <param name="data">The data.</param>
/// <returns></returns>
public static List<Type> ReadDialogs(string data)
{
return data.Split('\n')
.Select(x => x.Trim())
.Where(x => x.IsNotEmpty())
.Select(x => ManagedProject.GetDialog(x))
.ToList();
}
static void ValidateDialogInfo(string info)
{
try
{
GetDialog(info, true);
}
catch (Exception)
{
//may need to do extra logging; not important for now
throw;
}
}
static string GetDialogInfo(Type dialog)
{
var info = "{0}|{1}".FormatInline(
dialog.Assembly.FullName,
dialog.FullName);
return info;
}
internal static Type GetDialog(string info)
{
return GetDialog(info, false);
}
internal static Type GetDialog(string info, bool validate)
{
string[] parts = info.Split('|');
var assembly = System.Reflection.Assembly.Load(parts[0]);
if (validate)
ProjectValidator.ValidateAssemblyCompatibility(assembly);
return assembly.GetType(parts[1]);
}
static void ValidateHandlerInfo(string info)
{
try
{
GetHandler(info);
}
catch (Exception)
{
//may need to do extra logging; not important for now
throw;
}
}
internal static string GetHandlersInfo(MulticastDelegate handlers)
{
var result = new StringBuilder();
foreach (Delegate action in handlers.GetInvocationList())
{
var handlerInfo = "{0}|{1}|{2}".FormatInline(
action.Method.DeclaringType.Assembly.FullName,
action.Method.DeclaringType.FullName,
action.Method.Name);
ValidateHandlerInfo(handlerInfo);
result.AppendLine(handlerInfo);
}
return result.ToString().Trim();
}
static MethodInfo GetHandler(string info)
{
string[] parts = info.Split('|');
var assembly = System.Reflection.Assembly.Load(parts[0]);
Type type = null;
//Ideally need to iterate through the all types in order to find even private ones
//Though loading some internal (irrelevant) types can fail because of the dependencies.
try
{
assembly.GetTypes().Single(t => t.FullName == parts[1]);
}
catch { }
//If we failed to iterate through the types then try to load the type explicitly. Though in this case it has to be public.
if (type == null)
type = assembly.GetType(parts[1]);
var method = type.GetMethods(BindingFlags.Public | BindingFlags.NonPublic | BindingFlags.Instance | BindingFlags.InvokeMethod | BindingFlags.Static)
.Single(m => m.Name == parts[2]);
return method;
}
static void InvokeClientHandler(string info, SetupEventArgs eventArgs)
{
MethodInfo method = GetHandler(info);
if (method.IsStatic)
method.Invoke(null, new object[] { eventArgs });
else
method.Invoke(Activator.CreateInstance(method.DeclaringType), new object[] { eventArgs });
}
internal static ActionResult InvokeClientHandlers(Session session, string eventName, IShellView UIShell = null)
{
var eventArgs = Convert(session);
eventArgs.ManagedUIShell = UIShell;
try
{
string handlersInfo = session.Property("WixSharp_{0}_Handlers".FormatInline(eventName));
if (!string.IsNullOrEmpty(handlersInfo))
{
foreach (string item in handlersInfo.Trim().Split('\n'))
{
InvokeClientHandler(item.Trim(), eventArgs);
if (eventArgs.Result == ActionResult.Failure || eventArgs.Result == ActionResult.UserExit)
break;
}
eventArgs.SaveData();
}
}
catch (Exception e)
{
session.Log(e.Message);
}
return eventArgs.Result;
}
internal static ActionResult Init(Session session)
{
//System.Diagnostics.Debugger.Launch();
var data = new SetupEventArgs.AppData();
try
{
data["Installed"] = session["Installed"];
data["REMOVE"] = session["REMOVE"];
data["ProductName"] = session["ProductName"];
data["REINSTALL"] = session["REINSTALL"];
data["UPGRADINGPRODUCTCODE"] = session["UPGRADINGPRODUCTCODE"];
data["UILevel"] = session["UILevel"];
}
catch (Exception e)
{
session.Log(e.Message);
}
session["WIXSHARP_RUNTIME_DATA"] = data.ToString();
return ActionResult.Success;
}
static SetupEventArgs Convert(Session session)
{
//Debugger.Launch();
var result = new SetupEventArgs { Session = session };
try
{
string data = session.Property("WIXSHARP_RUNTIME_DATA");
result.Data.InitFrom(data);
}
catch (Exception e)
{
session.Log(e.Message);
}
return result;
}
}
}
| {
"content_hash": "1f34b8f4ac25cd84cc0360bc80f2f2bc",
"timestamp": "",
"source": "github",
"line_count": 438,
"max_line_length": 167,
"avg_line_length": 39.84931506849315,
"alnum_prop": 0.5598716626561246,
"repo_name": "Eun/TestRepo",
"id": "0096b5d7a39cdc83702551c834e41ab106a0e060",
"size": "17454",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "src/WixSharp/ManagedProject/ManagedProject.cs",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "ASP",
"bytes": "932"
},
{
"name": "Batchfile",
"bytes": "13179"
},
{
"name": "C#",
"bytes": "1830314"
},
{
"name": "CSS",
"bytes": "1775"
},
{
"name": "HTML",
"bytes": "3914"
},
{
"name": "Smalltalk",
"bytes": "8444"
},
{
"name": "Visual Basic",
"bytes": "136"
}
],
"symlink_target": ""
} |
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace DatabaseV3
{
/// <summary>
/// Represents an array in a document.
/// </summary>
public class DocumentArray : IDocumentElement
{
/// <summary>
/// The data in the array.
/// </summary>
private List<IDocumentElement> _data = new List<IDocumentElement>();
/// <summary>
/// Initializes a new instance of the <see cref="DocumentArray"/> class.
/// </summary>
/// <param name="data">The data in the array.</param>
public DocumentArray(IEnumerable<object> data)
{
foreach (var item in data)
{
if (item is IDocumentElement)
{
_data.Add((IDocumentElement)item);
}
else
{
_data.Add(new DocumentElement(item));
}
}
}
/// <inheritdoc />
public bool? AsBool
{
get
{
return null;
}
}
/// <inheritdoc />
public Document AsDocument
{
get
{
return null;
}
}
/// <inheritdoc />
public DocumentArray AsDocumentArray
{
get
{
return this;
}
}
/// <inheritdoc />
public int? AsInt32
{
get
{
return null;
}
}
/// <inheritdoc />
public long? AsInt64
{
get
{
return null;
}
}
/// <inheritdoc />
public string AsString
{
get
{
return null;
}
}
/// <summary>
/// Gets the value at the specified index.
/// </summary>
/// <param name="i">The index to get.</param>
/// <returns>The value at the specified index.</returns>
public IDocumentElement this[int i]
{
get
{
return _data[i];
}
}
/// <inheritdoc />
public override bool Equals(object obj)
{
DocumentArray array = obj as DocumentArray;
return array != null && _data.Count == array._data.Count && _data.Except(array._data).Any();
}
/// <inheritdoc />
public override int GetHashCode()
{
return _data.GetHashCode();
}
/// <inheritdoc />
public override string ToString()
{
StringBuilder builder = new StringBuilder();
builder.Append("[");
bool first = true;
foreach (var item in _data)
{
if (!first)
{
builder.Append(",");
}
else
{
first = false;
}
builder.Append(item);
}
builder.Append("]");
return builder.ToString();
}
}
} | {
"content_hash": "d5790527e1bb00f066a191cd3fa0666c",
"timestamp": "",
"source": "github",
"line_count": 140,
"max_line_length": 104,
"avg_line_length": 23.1,
"alnum_prop": 0.4072356215213358,
"repo_name": "CaptainCow95/DatabaseV3",
"id": "f4de244127a4e6d82d9fa4d2c3f586f268ea2c79",
"size": "3236",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "DatabaseV3/DocumentArray.cs",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "C#",
"bytes": "46946"
}
],
"symlink_target": ""
} |
package org.apache.catalina.webresources;
import java.io.File;
import org.junit.Assert;
import org.junit.Test;
import org.apache.catalina.Context;
import org.apache.catalina.WebResource;
import org.apache.catalina.core.StandardHost;
import org.apache.catalina.startup.Tomcat;
import org.apache.catalina.startup.TomcatBaseTest;
public class TestAbstractArchiveResource extends TomcatBaseTest {
@Test
public void testNestedJarGetURL() throws Exception {
Tomcat tomcat = getTomcatInstance();
File docBase = new File("test/webresources/war-url-connection.war");
Context ctx = tomcat.addWebapp("/test", docBase.getAbsolutePath());
skipTldsForResourceJars(ctx);
((StandardHost) tomcat.getHost()).setUnpackWARs(false);
tomcat.start();
WebResource webResource =
ctx.getResources().getClassLoaderResource("/META-INF/resources/index.html");
StringBuilder expectedURL = new StringBuilder("jar:war:");
expectedURL.append(docBase.getAbsoluteFile().toURI().toURL().toString());
expectedURL.append("*/WEB-INF/lib/test.jar!/META-INF/resources/index.html");
Assert.assertEquals(expectedURL.toString(), webResource.getURL().toString());
}
@Test
public void testJarGetURL() throws Exception {
Tomcat tomcat = getTomcatInstance();
File docBase = new File("test/webapp");
Context ctx = tomcat.addWebapp("/test", docBase.getAbsolutePath());
skipTldsForResourceJars(ctx);
((StandardHost) tomcat.getHost()).setUnpackWARs(false);
tomcat.start();
WebResource webResource =
ctx.getResources().getClassLoaderResource("/META-INF/tags/echo.tag");
StringBuilder expectedURL = new StringBuilder("jar:");
expectedURL.append(docBase.getAbsoluteFile().toURI().toURL().toString());
expectedURL.append("WEB-INF/lib/test-lib.jar!/META-INF/tags/echo.tag");
Assert.assertEquals(expectedURL.toString(), webResource.getURL().toString());
}
}
| {
"content_hash": "afbc31510bd1c35bf1dafd2e2ca882db",
"timestamp": "",
"source": "github",
"line_count": 62,
"max_line_length": 92,
"avg_line_length": 34.11290322580645,
"alnum_prop": 0.6728132387706856,
"repo_name": "IAMTJW/Tomcat-8.5.20",
"id": "60984e50d03c605a58c5a169c49442928e11b9e0",
"size": "2932",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "tomcat-8.5.20/test/org/apache/catalina/webresources/TestAbstractArchiveResource.java",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "Batchfile",
"bytes": "38058"
},
{
"name": "CSS",
"bytes": "14009"
},
{
"name": "HTML",
"bytes": "333233"
},
{
"name": "Java",
"bytes": "19675734"
},
{
"name": "NSIS",
"bytes": "44042"
},
{
"name": "Perl",
"bytes": "14275"
},
{
"name": "Shell",
"bytes": "48396"
},
{
"name": "XSLT",
"bytes": "32480"
}
],
"symlink_target": ""
} |
define(['durandal/plugins/router'], function (router) {
return {
router: router,
activate: function () {
router.map([
{ url: 'hello', moduleId: 'samples/hello/index', name: 'Hello World', visible: true },
{ url: 'view-composition', moduleId: 'samples/viewComposition/index', name: 'View Composition', visible: true },
{ url: 'modal', moduleId: 'samples/modal/index', name: 'Modal Dialogs', visible: true },
{ url: 'event-aggregator', moduleId: 'samples/eventAggregator/index', name: 'Events', visible: true },
{ url: 'widgets', moduleId: 'samples/widgets/index', name: 'Widgets', visible: true },
{ url: 'master-detail', moduleId: 'samples/masterDetail/index', name: 'Master Detail', visible: true },
{ url: 'knockout-samples/:name', moduleId: 'samples/knockout/index', name: 'Knockout Samples' },
{ url: 'knockout-samples', moduleId: 'samples/knockout/index', name: 'Knockout Samples', visible: true }
]);
return router.activate('hello');
}
};
}); | {
"content_hash": "3934aa226e5a912a7156e6b432790182",
"timestamp": "",
"source": "github",
"line_count": 20,
"max_line_length": 128,
"avg_line_length": 58.15,
"alnum_prop": 0.5692175408426483,
"repo_name": "robksawyer/durandaljs",
"id": "03de97492c222cc6eabc169c1503a06376da2a9c",
"size": "1165",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "example/samples/shell.js",
"mode": "33261",
"license": "mit",
"language": [
{
"name": "JavaScript",
"bytes": "197408"
}
],
"symlink_target": ""
} |
package com.netflix.hollow.core.index;
import static com.netflix.hollow.core.schema.HollowObjectSchema.FieldType;
import static com.netflix.hollow.core.schema.HollowSchema.SchemaType;
import com.netflix.hollow.core.read.dataaccess.HollowCollectionTypeDataAccess;
import com.netflix.hollow.core.read.dataaccess.HollowDataAccess;
import com.netflix.hollow.core.read.dataaccess.HollowMapTypeDataAccess;
import com.netflix.hollow.core.read.dataaccess.HollowObjectTypeDataAccess;
import com.netflix.hollow.core.read.dataaccess.HollowTypeDataAccess;
import com.netflix.hollow.core.read.iterator.HollowMapEntryOrdinalIterator;
import com.netflix.hollow.core.read.iterator.HollowOrdinalIterator;
import com.netflix.hollow.core.schema.HollowCollectionSchema;
import com.netflix.hollow.core.schema.HollowMapSchema;
import com.netflix.hollow.core.schema.HollowObjectSchema;
import com.netflix.hollow.core.schema.HollowSchema;
import java.util.ArrayList;
import java.util.List;
/**
* This class is used to represent a field path. A field path is a "." separated list of fields that are used for traversal when looking up values for a type and ordinal of that type.
* It is used to read field values for a given type and its ordinal. It is convenient, when the path is deeply nested.
* <p>
* Upon initializing a instance of this class with given parameters, it does the following checks
* <ul>
* <li>validates the path by traversing it and making sure all types are present in the HollowDataAccess</li>
* <li>auto-expands the path for collections (appending ".element" if missing), and a reference type (e.g String/Integer appending ".value" if missing) with single field. (except for Map schema)</li>
* <li>for map schema type, the class looks for "key" to iterate over key types and "value" to iterate over value types.</li>
* </ul>
* <p>
* This class has a convenience method to find values following the field path and given a start field position.
*/
class FieldPath {
private final String fieldPath;
private final HollowDataAccess hollowDataAccess;
private final String type;
private String[] fields;
private int[] fieldPositions;
private FieldType[] fieldTypes;
private String lastRefTypeInPath;
private boolean autoExpand;
/**
* Create new FieldPath with auto-expand feature.
*
* @param hollowDataAccess hollow data access
* @param type parent type of the field path
* @param fieldPath "." separated fields
*/
FieldPath(HollowDataAccess hollowDataAccess, String type, String fieldPath) {
this(hollowDataAccess, type, fieldPath, true);
}
/**
* Create new FieldPath.
*
* @param hollowDataAccess hollow data access
* @param type parent type of the field path
* @param fieldPath "." separated fields
* @param autoExpand if the field path should be auto-expand collections and references with one field.
*/
FieldPath(HollowDataAccess hollowDataAccess, String type, String fieldPath, boolean autoExpand) {
this.fieldPath = fieldPath;
this.hollowDataAccess = hollowDataAccess;
this.type = type;
this.autoExpand = autoExpand;
initialize();
}
private void initialize() {
FieldPaths.FieldPath<FieldPaths.FieldSegment> path =
FieldPaths.createFieldPathForPrefixIndex(hollowDataAccess, type, fieldPath, autoExpand);
List<String> fields = new ArrayList<>();
List<Integer> fieldPositions = new ArrayList<>();
List<FieldType> fieldTypes = new ArrayList<>();
String lastRefType = type;
for (FieldPaths.FieldSegment segment : path.getSegments()) {
fields.add(segment.getName());
if (segment.getEnclosingSchema().getSchemaType() == SchemaType.OBJECT) {
assert segment instanceof FieldPaths.ObjectFieldSegment;
FieldPaths.ObjectFieldSegment oSegment = (FieldPaths.ObjectFieldSegment) segment;
fieldPositions.add(oSegment.getIndex());
fieldTypes.add(oSegment.getType());
} else {
fieldPositions.add(0);
fieldTypes.add(FieldType.REFERENCE);
}
String refType = segment.getTypeName();
if (refType != null) {
lastRefType = refType;
}
}
this.fields = fields.toArray(new String[0]);
this.fieldPositions = fieldPositions.stream().mapToInt(i -> i).toArray();
this.fieldTypes = fieldTypes.toArray(new FieldType[0]);
this.lastRefTypeInPath = lastRefType;
}
String getLastRefTypeInPath() {
return lastRefTypeInPath;
}
FieldType getLastFieldType() {
return fieldTypes[this.fields.length - 1];
}
/**
* Recursively find all the values following the field path.
*
* @param ordinal ordinal record for the given type in field path
* @return Array of values found at the field path for the given ordinal record in the type.
*/
Object[] findValues(int ordinal) {
return getAllValues(ordinal, type, 0);
}
/**
* Recursively find a value following the path. If the path contains a collection, then the first value is picked.
*
* @param ordinal the ordinal used to find a value
* @return A value found at the field path for the given ordinal record in the type.
*/
Object findValue(int ordinal) {
return getValue(ordinal, type, 0);
}
private Object getValue(int ordinal, String type, int fieldIndex) {
Object value = null;
HollowTypeDataAccess typeDataAccess = hollowDataAccess.getTypeDataAccess(type);
SchemaType schemaType = hollowDataAccess.getSchema(type).getSchemaType();
HollowSchema schema = hollowDataAccess.getSchema(type);
if (schemaType.equals(SchemaType.LIST) || schemaType.equals(SchemaType.SET)) {
HollowCollectionTypeDataAccess collectionTypeDataAccess = (HollowCollectionTypeDataAccess) typeDataAccess;
HollowCollectionSchema collectionSchema = (HollowCollectionSchema) schema;
String elementType = collectionSchema.getElementType();
HollowOrdinalIterator it = collectionTypeDataAccess.ordinalIterator(ordinal);
int refOrdinal = it.next();
if (refOrdinal != HollowOrdinalIterator.NO_MORE_ORDINALS) {
value = getValue(refOrdinal, elementType, fieldIndex + 1);
}
return value;
} else if (schemaType.equals(SchemaType.MAP)) {
// Map type
HollowMapTypeDataAccess mapTypeDataAccess = (HollowMapTypeDataAccess) typeDataAccess;
HollowMapSchema mapSchema = (HollowMapSchema) schema;
// what to iterate on in map
boolean iterateThroughKeys = fields[fieldIndex].equals("key");
String keyOrValueType = iterateThroughKeys ? mapSchema.getKeyType() : mapSchema.getValueType();
HollowMapEntryOrdinalIterator mapEntryIterator = mapTypeDataAccess.ordinalIterator(ordinal);
if (mapEntryIterator.next()) {
int keyOrValueOrdinal = iterateThroughKeys ? mapEntryIterator.getKey() : mapEntryIterator.getValue();
value = getValue(keyOrValueOrdinal, keyOrValueType, fieldIndex + 1);
}
return value;
} else {
// Object type
HollowObjectSchema objectSchema = (HollowObjectSchema) schema;
HollowObjectTypeDataAccess objectTypeDataAccess = (HollowObjectTypeDataAccess) typeDataAccess;
if (fieldTypes[fieldIndex].equals(FieldType.REFERENCE)) {
int refOrdinal = objectTypeDataAccess.readOrdinal(ordinal, fieldPositions[fieldIndex]);
if (refOrdinal >= 0) {
String refType = objectSchema.getReferencedType(fieldPositions[fieldIndex]);
value = getValue(refOrdinal, refType, fieldIndex + 1);
}
} else {
value = readFromObject(objectTypeDataAccess, ordinal, fieldTypes[fieldIndex], fieldPositions[fieldIndex]);
}
}
return value;
}
private Object[] getAllValues(int ordinal, String type, int fieldIndex) {
Object[] values;
HollowTypeDataAccess typeDataAccess = hollowDataAccess.getTypeDataAccess(type);
SchemaType schemaType = hollowDataAccess.getSchema(type).getSchemaType();
HollowSchema schema = hollowDataAccess.getSchema(type);
if (schemaType.equals(SchemaType.LIST) || schemaType.equals(SchemaType.SET)) {
HollowCollectionTypeDataAccess collectionTypeDataAccess = (HollowCollectionTypeDataAccess) typeDataAccess;
HollowCollectionSchema collectionSchema = (HollowCollectionSchema) schema;
String elementType = collectionSchema.getElementType();
HollowOrdinalIterator it = collectionTypeDataAccess.ordinalIterator(ordinal);
List<Object> valueList = new ArrayList<>();
int refOrdinal = it.next();
while (refOrdinal != HollowOrdinalIterator.NO_MORE_ORDINALS) {
Object[] refValues = getAllValues(refOrdinal, elementType, fieldIndex + 1);
for (Object value : refValues)
valueList.add(value);
refOrdinal = it.next();
}
values = new Object[valueList.size()];
valueList.toArray(values);
} else if (schemaType.equals(SchemaType.MAP)) {
// Map type
HollowMapTypeDataAccess mapTypeDataAccess = (HollowMapTypeDataAccess) typeDataAccess;
HollowMapSchema mapSchema = (HollowMapSchema) schema;
// what to iterate on in map
boolean iterateThroughKeys = fields[fieldIndex].equals("key");
String keyOrValueType = iterateThroughKeys ? mapSchema.getKeyType() : mapSchema.getValueType();
HollowMapEntryOrdinalIterator mapEntryIterator = mapTypeDataAccess.ordinalIterator(ordinal);
List<Object> valueList = new ArrayList<>();
while (mapEntryIterator.next()) {
int keyOrValueOrdinal = iterateThroughKeys ? mapEntryIterator.getKey() : mapEntryIterator.getValue();
Object[] refValues = getAllValues(keyOrValueOrdinal, keyOrValueType, fieldIndex + 1);
for (Object value : refValues)
valueList.add(value);
}
values = new Object[valueList.size()];
valueList.toArray(values);
} else {
// Object type
HollowObjectSchema objectSchema = (HollowObjectSchema) schema;
HollowObjectTypeDataAccess objectTypeDataAccess = (HollowObjectTypeDataAccess) typeDataAccess;
if (fieldTypes[fieldIndex].equals(FieldType.REFERENCE)) {
int refOrdinal = objectTypeDataAccess.readOrdinal(ordinal, fieldPositions[fieldIndex]);
if (refOrdinal >= 0) {
String refType = objectSchema.getReferencedType(fieldPositions[fieldIndex]);
return getAllValues(refOrdinal, refType, fieldIndex + 1);
}
return new Object[]{};
} else {
return new Object[]{readFromObject(objectTypeDataAccess, ordinal, fieldTypes[fieldIndex], fieldPositions[fieldIndex])};
}
}
return values;
}
private Object readFromObject(HollowObjectTypeDataAccess objectTypeDataAccess, int ordinal, FieldType fieldType, int fieldPosition) {
Object value;
switch (fieldType) {
case INT:
value = objectTypeDataAccess.readInt(ordinal, fieldPosition);
break;
case LONG:
value = objectTypeDataAccess.readLong(ordinal, fieldPosition);
break;
case DOUBLE:
value = objectTypeDataAccess.readDouble(ordinal, fieldPosition);
break;
case FLOAT:
value = objectTypeDataAccess.readFloat(ordinal, fieldPosition);
break;
case BOOLEAN:
value = objectTypeDataAccess.readBoolean(ordinal, fieldPosition);
break;
case STRING:
value = objectTypeDataAccess.readString(ordinal, fieldPosition);
break;
default:
throw new IllegalStateException("Invalid field type :" + fieldType + " cannot read values for this type");
}
return value;
}
}
| {
"content_hash": "af695b56117eb699b622aca694300399",
"timestamp": "",
"source": "github",
"line_count": 285,
"max_line_length": 199,
"avg_line_length": 44.46666666666667,
"alnum_prop": 0.6601436124043242,
"repo_name": "Netflix/hollow",
"id": "2ee298fed5a2d2c0ad8dfcce15803abe6ca6c87b",
"size": "13314",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "hollow/src/main/java/com/netflix/hollow/core/index/FieldPath.java",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "CSS",
"bytes": "4299"
},
{
"name": "Java",
"bytes": "4694590"
},
{
"name": "JavaScript",
"bytes": "1703"
},
{
"name": "Shell",
"bytes": "1730"
}
],
"symlink_target": ""
} |
<?xml version="1.0" encoding="utf-8"?>
<layout xmlns:android="http://schemas.android.com/apk/res/android">
<RelativeLayout xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/activity_splash"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.example.jingbin.cloudreader.SplashActivity">
<com.youth.banner.Banner
android:id="@+id/banner_splash"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:image_scale_type="fit_xy"
app:indicator_drawable_selected="@drawable/banner_red"
app:indicator_drawable_unselected="@drawable/banner_grey"
app:indicator_height="9dp"
app:indicator_margin="3dp"
app:indicator_width="9dp"
app:is_auto_play="false" />
<!--<com.daimajia.slider.library.SliderLayout xmlns:custom="http://schemas.android.com/apk/res-auto"-->
<!--android:id="@+id/slider"-->
<!--android:layout_width="match_parent"-->
<!--android:layout_height="match_parent"-->
<!--custom:auto_cycle="false"-->
<!--custom:indicator_visibility="visible"-->
<!--custom:pager_animation="Default"-->
<!--custom:pager_animation_span="1100" />-->
<TextView
android:layout_width="170dp"
android:layout_height="40dp"
android:layout_alignParentBottom="true"
android:layout_centerHorizontal="true"
android:layout_marginBottom="30dp"
android:background="@drawable/home_adjust_background"
android:gravity="center"
android:text="立即体验"
android:textColor="@color/colorTheme"
android:textSize="16sp" />
</RelativeLayout>
</layout>
| {
"content_hash": "264b7a42832f88cc1424bd85f3f9a604",
"timestamp": "",
"source": "github",
"line_count": 44,
"max_line_length": 111,
"avg_line_length": 43.31818181818182,
"alnum_prop": 0.6091290661070304,
"repo_name": "weiwenqiang/GitHub",
"id": "05f412c91ef96056d663594aafb349731e83ab3d",
"size": "1914",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "MVVM/CloudReader-master/app/src/main/res/layout/activity_splash.xml",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "Batchfile",
"bytes": "87"
},
{
"name": "C",
"bytes": "42062"
},
{
"name": "C++",
"bytes": "12137"
},
{
"name": "CMake",
"bytes": "202"
},
{
"name": "CSS",
"bytes": "75087"
},
{
"name": "Clojure",
"bytes": "12036"
},
{
"name": "FreeMarker",
"bytes": "21704"
},
{
"name": "Groovy",
"bytes": "55083"
},
{
"name": "HTML",
"bytes": "61549"
},
{
"name": "Java",
"bytes": "42222825"
},
{
"name": "JavaScript",
"bytes": "216823"
},
{
"name": "Kotlin",
"bytes": "24319"
},
{
"name": "Makefile",
"bytes": "19490"
},
{
"name": "Perl",
"bytes": "280"
},
{
"name": "Prolog",
"bytes": "1030"
},
{
"name": "Python",
"bytes": "13032"
},
{
"name": "Scala",
"bytes": "310450"
},
{
"name": "Shell",
"bytes": "27802"
}
],
"symlink_target": ""
} |
class Create<%= class_name %> < ActiveRecord::Migration
def self.up
create_table :<%= table_name %> do |t|
t.string :event_type
t.references :object, :polymorphic => true
t.references :user
t.timestamps
end
add_index :<%= table_name %>, :event_type
add_index :<%= table_name %>, [:object_type, :object_id]
add_index :<%= table_name %>, :user_id
end
def self.down
drop_table :<%= table_name %>
end
end
| {
"content_hash": "d90a1825f190fe1dde2fbb96693b798c",
"timestamp": "",
"source": "github",
"line_count": 18,
"max_line_length": 60,
"avg_line_length": 25.5,
"alnum_prop": 0.5925925925925926,
"repo_name": "tomaass/billapp_event_horizon",
"id": "ce7adddd18444f2cc4cd0b6a4928c3a6001d67e7",
"size": "459",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "lib/generators/event_migration/templates/migration.rb",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "Ruby",
"bytes": "3640"
}
],
"symlink_target": ""
} |
require 'rake'
require 'pp'
require 'yaml'
require 'rubygems'
require 'stackbuilder/stacks/environment'
require 'stackbuilder/stacks/inventory'
require 'stackbuilder/support/mcollective'
require 'stackbuilder/support/mcollective_puppet'
require 'stackbuilder/compute/controller'
require 'stackbuilder/allocator/host_repository'
require 'stackbuilder/allocator/host_preference'
require 'stackbuilder/allocator/host_policies'
require 'stackbuilder/allocator/ephemeral_allocator'
require 'stackbuilder/dns/basic_dns_service'
require 'stackbuilder/stacks/core/services'
require 'stackbuilder/stacks/namespace'
class Stacks::Factory
attr_reader :path
attr_reader :inventory
def initialize(inventory, path = nil, ignore_spectre_patching_host_policy = false)
@path = path
@inventory = inventory
@ignore_spectre_patching_host_policy = ignore_spectre_patching_host_policy
end
def policies
if defined? @policies
@policies
else
policies = [
StackBuilder::Allocator::HostPolicies.allocate_on_host_with_tags,
StackBuilder::Allocator::HostPolicies.ensure_mount_points_have_specified_storage_types_policy,
StackBuilder::Allocator::HostPolicies.ensure_defined_storage_types_policy,
StackBuilder::Allocator::HostPolicies.do_not_overallocate_disk_policy,
StackBuilder::Allocator::HostPolicies.ha_group,
StackBuilder::Allocator::HostPolicies.do_not_overallocate_ram_policy,
StackBuilder::Allocator::HostPolicies.allocation_temporarily_disabled_policy,
StackBuilder::Allocator::HostPolicies.require_persistent_storage_to_exist_policy
]
if !@ignore_spectre_patching_host_policy
policies.push(StackBuilder::Allocator::HostPolicies.spectre_patch_status_of_vm_must_match_spectre_patch_status_of_host_policy)
end
policies
end
end
def preference_functions
@preference_functions ||= [
StackBuilder::Allocator::HostPreference.prefer_not_g9,
StackBuilder::Allocator::HostPreference.prefer_no_data,
StackBuilder::Allocator::HostPreference.fewest_machines,
StackBuilder::Allocator::HostPreference.prefer_diverse_vm_rack_distribution,
StackBuilder::Allocator::HostPreference.alphabetical_fqdn
]
end
def compute_controller
@compute_controller ||= Compute::Controller.new
end
def compute_node_client
@compute_node_client ||= Compute::Client.new
end
def dns_service
@dns_service ||= StackBuilder::DNS::BasicDNSService.new
end
def host_repository
@host_repository ||= StackBuilder::Allocator::HostRepository.new(
:machine_repo => inventory,
:preference_functions => preference_functions,
:policies => policies,
:compute_node_client => compute_node_client
)
end
def allocator
StackBuilder::Allocator::EphemeralAllocator.new(:host_repository => host_repository)
end
def services
@services ||= Stacks::Core::Services.new(
:compute_controller => compute_controller,
:allocator => allocator,
:dns_service => dns_service
)
end
def refresh(validate = true)
@inventory = Stacks::Inventory.from_dir(@path, validate)
end
end
| {
"content_hash": "bed2fab68e3f4a281a5403a8fa6c79fd",
"timestamp": "",
"source": "github",
"line_count": 95,
"max_line_length": 134,
"avg_line_length": 33.88421052631579,
"alnum_prop": 0.7291084187635912,
"repo_name": "tim-group/stackbuilder",
"id": "5923ad2fcafc2abc1e4149d96e50db66346e0d16",
"size": "3219",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "lib/stackbuilder/stacks/factory.rb",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "Dockerfile",
"bytes": "1187"
},
{
"name": "Ruby",
"bytes": "1089014"
},
{
"name": "Shell",
"bytes": "9993"
}
],
"symlink_target": ""
} |
(function () {
angular.module('sp-peoplepicker', []).directive('spPeoplePicker', function ($q, $timeout, $compile) {
//Usage:
//<sp-people-picker name="taskAssignee" id="taskAssignee" data-ng-model="$scope.taskAssignees" min-entries="1" max-entries="5" allow-duplicates="false" show-login="false" show-title="true" min-characters="2" app-web-url="$scope.spAppWebUrl" />
var directive = {
link: link,
restrict: 'EA',
require: 'ngModel',
replace: true,
scope: {
minEntries: '@',
maxEntries: '@',
allowDuplicates: '@',
showLogin: '=',
showTitle: '=',
minCharacters: '@',
selections: '=',
appWebUrl: '='
},
template: function (element, attrs) {
var htmlText =
'<div class="cam-peoplepicker-input">' +
'<div id="divPeoplePick" class="cam-peoplepicker-userlookup ms-fullWidth">' +
'<span id="spanPeoplePick"></span>' +
'<input type="text" id="inputPeoplePick" class="cam-peoplepicker-edit" />' +
'</div>' +
'<div id="divPeoplePickSearch" class="cam-peoplepicker-usersearch ms-emphasisBorder"></div>' +
'<input type="hidden" name="hdnPeoplePick" id="hdnPeoplePick" />' +
'</div>';
return htmlText;
},
controller: ['$scope', '$element', function ($scope, $element) {
$scope.spContext = new SP.ClientContext($scope.appWebUrl);
var factory = new SP.ProxyWebRequestExecutorFactory($scope.appWebUrl);
$scope.spContext.set_webRequestExecutorFactory(factory);
$scope.peoplePicker = new CAMControl.PeoplePicker($scope.spContext, $('#spanPeoplePick', $element), $('#inputPeoplePick', $element), $('#divPeoplePickSearch', $element), $('#hdnPeoplePick', $element));
$scope.peoplePicker.$scope = $scope;
$scope.peoplePicker.$compile = $compile;
}]
};
return directive;
function link(scope, element, attrs, ngModel) {
//Make a people picker control
$q.when().then(function() {
var deferred = $q.defer();
$timeout(function() {
var value = (attrs.value || ngModel.$modelValue || ngModel.$viewValue );
if (value) {
$('#hdnPeoplePick', element).val(typeof(value) === 'string' ? value : JSON.stringify(value));
}
deferred.resolve();
});
return deferred.promise;
}).then(function() {
//1. scope.spContext = SharePoint Client Context object
//2. $('#spanPeoplePick') = SPAN that will 'host' the people picker control
//3. $('#inputPeoplePick') = INPUT that will be used to capture user input
//4. $('#divPeoplePickSearch') = DIV that will show the 'dropdown' of the people picker
//5. $('#hdnPeoplePick') = INPUT hidden control that will host a JSON string of the resolved users
// required to pass the variable name here!
scope.peoplePicker.InstanceName = "peoplePicker";
// Pass current language, if not set defaults to en-US. Use the SPLanguage query string param or provide a string like "nl-BE"
// Do not set the Language property if you do not have foreseen javascript resource file for your language
//peoplePicker.Language = spLanguage;
// optionally show more/less entries in the people picker dropdown, 4 is the default
scope.peoplePicker.MaxEntriesShown = scope.maxEntries;
// Can duplicate entries be selected (default = false)
scope.peoplePicker.AllowDuplicates = scope.allowDuplicates;
// Show the user loginname
scope.peoplePicker.ShowLoginName = scope.showLogin;
// Show the user title
scope.peoplePicker.ShowTitle = scope.showTitle;
// Invoke a method when a new recipient is selected
scope.peoplePicker.OnSelectionChanged = function () {
var result = JSON.parse($('#hdnPeoplePick', element).val());
ngModel.$setViewValue(result);
ngModel.$render();
scope.$apply();
// check validity
isValid();
};
// Set principal type to determine what is shown (default = 1, only users are resolved).
// See http://msdn.microsoft.com/en-us/library/office/microsoft.sharepoint.client.utilities.principaltype.aspx for more details
// Set ShowLoginName and ShowTitle to false if you're resolving groups
scope.peoplePicker.PrincipalType = SP.Utilities.PrincipalType.all;
// start user resolving as of 2 entered characters (= default)
scope.peoplePicker.MinimalCharactersBeforeSearching = scope.minCharacters;
// Hookup everything
scope.peoplePicker.Initialize();
isValid();
// check if the field is valid
function isValid() {
var minBounds = true;
var maxBounds = true;
if(ngModel.$viewValue) {
if (attrs.hasOwnProperty('minEntries')) {
minBounds = (ngModel.$viewValue.length >= parseInt(scope.minEntries, 10));
}
if (attrs.hasOwnProperty('maxEntries')) {
maxBounds = (ngModel.$viewValue.length <= parseInt(scope.maxEntries, 10));
}
}
var valid = minBounds && maxBounds;
ngModel.$setValidity('required', valid);
}
});
}
});
var CAMControl;
(function (CAMControl) {
var PeoplePicker = (function () {
// Constructor
function PeoplePicker(SharePointContext, PeoplePickerControl, PeoplePickerEdit, PeoplePickerDisplay, PeoplePickerData) {
//public properties
this.SharePointContext = SharePointContext;
this.PeoplePickerControl = PeoplePickerControl;
this.PeoplePickerEdit = PeoplePickerEdit;
this.PeoplePickerDisplay = PeoplePickerDisplay;
this.PeoplePickerData = PeoplePickerData;
this.InstanceName = "";
this.MaxEntriesShown = 4;
this.ShowLoginName = true;
this.ShowTitle = true;
this.MinimalCharactersBeforeSearching = 2;
this.PrincipalType = 1;
this.AllowDuplicates = false;
this.Language = "en-us";
this.OnSelectionChanged = null;
//Private variable is not really private, just a naming convention
this._queryID = 1;
this._lastQueryID = 1;
this._ResolvedUsers = [];
this.$scope = null;
this.$compile = null;
}
// Property wrapped in function to allow access from event handler
PeoplePicker.prototype.GetPrincipalType = function () {
return this.PrincipalType;
};
// Property wrapped in function to allow access from event handler
PeoplePicker.prototype.SetPrincipalType = function (principalType) {
//See http://msdn.microsoft.com/en-us/library/office/microsoft.sharepoint.client.utilities.principaltype.aspx
//This enumeration has a FlagsAttribute attribute that allows a bitwise combination of its member values.
//None Enumeration whose value specifies no principal type. Value = 0.
//User Enumeration whose value specifies a user as the principal type. Value = 1.
//DistributionList Enumeration whose value specifies a distribution list as the principal type. Value = 2.
//SecurityGroup Enumeration whose value specifies a security group as the principal type. Value = 4.
//SharePointGroup Enumeration whose value specifies a group (2) as the principal type. Value = 8.
//All Enumeration whose value specifies all principal types. Value = 15.
this.PrincipalType = principalType;
};
// Property wrapped in function to allow access from event handler
PeoplePicker.prototype.GetMinimalCharactersBeforeSearching = function () {
return this.MinimalCharactersBeforeSearching;
};
// Property wrapped in function to allow access from event handler
PeoplePicker.prototype.SetMinimalCharactersBeforeSearching = function (minimalChars) {
this.MinimalCharactersBeforeSearching = minimalChars;
};
// HTML encoder
PeoplePicker.prototype.HtmlEncode = function (html) {
return document.createElement('a').appendChild(document.createTextNode(html)).parentNode.innerHTML;
};
// HTML decoder
PeoplePicker.prototype.HtmlDecode = function (html) {
var a = document.createElement('a');
a.innerHTML = html;
return a.textContent;
};
// Replace all string occurances, add a bew ReplaceAll method to the string type
String.prototype.ReplaceAll = function (token, newToken, ignoreCase) {
var _token;
var str = this + "";
var i = -1;
if (typeof token === "string") {
if (ignoreCase) {
_token = token.toLowerCase();
while ((
i = str.toLowerCase().indexOf(
token, i >= 0 ? i + newToken.length : 0
)) !== -1
) {
str = str.substring(0, i) +
newToken +
str.substring(i + token.length);
}
} else {
return this.split(token).join(newToken);
}
}
return str;
};
PeoplePicker.prototype.LoadScript = function (url, callback) {
var head = document.getElementsByTagName("head")[0];
var script = document.createElement("script");
script.src = url;
// Attach handlers for all browsers
var done = false;
script.onload = script.onreadystatechange = function () {
if (!done && (!this.readyState
|| this.readyState == "loaded"
|| this.readyState == "complete")) {
done = true;
// Continue your code
callback();
// Handle memory leak in IE
script.onload = script.onreadystatechange = null;
head.removeChild(script);
}
};
head.appendChild(script);
};
// String formatting
PeoplePicker.prototype.Format = function (str) {
for (var i = 1; i < arguments.length; i++) {
str = str.ReplaceAll("{" + (i - 1) + "}", arguments[i]);
}
return str;
};
// Hide the user selection box
PeoplePicker.prototype.HideSelectionBox = function () {
this.PeoplePickerDisplay.css('display', 'none');
};
// show the user selection box
PeoplePicker.prototype.ShowSelectionBox = function () {
this.PeoplePickerDisplay.css('display', 'block');
};
// Generates the html for a resolved user
PeoplePicker.prototype.ConstructResolvedUserSpan = function (login, name, lookupId) {
resultDisplay = 'Remove person or group {0}';
if (typeof deleteUser != 'undefined') {
resultDisplay = deleteUser;
}
lookupValue = (login) ? login.replace("\\", "\\\\") : lookupId;
resultDisplay = this.Format(resultDisplay, name);
userDisplaySpanTemplate = '<span class="cam-peoplepicker-userSpan"><span class="cam-entity-resolved">{0}</span><a title="{3}" class="cam-peoplepicker-delImage" ng-click="{1}.DeleteProcessedUser({2})" href>x</a></span>';
return this.Format(userDisplaySpanTemplate, name, this.InstanceName, "'" + lookupValue + "'", resultDisplay);
};
// Create a html representation of the resolved user array
PeoplePicker.prototype.ResolvedUsersToHtml = function () {
var userHtml = '';
for (var i = 0; i < this._ResolvedUsers.length; i++) {
userHtml += this.ConstructResolvedUserSpan(this._ResolvedUsers[i].Login, this._ResolvedUsers[i].Name, this._ResolvedUsers[i].LookupId);
}
return this.$compile(userHtml)(this.$scope);
};
// Returns a resolved user object
PeoplePicker.prototype.ResolvedUser = function (login, name, email) {
var user = {};
user.Login = login;
user.Name = name;
user.Email = email;
return user;
};
// Add resolved user to array and updates the hidden field control with a JSON string
PeoplePicker.prototype.PushResolvedUser = function (resolvedUser) {
if (this.AllowDuplicates) {
this._ResolvedUsers.push(resolvedUser);
} else {
var duplicate = false;
for (var i = 0; i < this._ResolvedUsers.length; i++) {
if (this._ResolvedUsers[i].Login == resolvedUser.Login) {
duplicate = true;
}
}
if (!duplicate) {
this._ResolvedUsers.push(resolvedUser);
}
}
this.PeoplePickerData.val(JSON.stringify(this._ResolvedUsers));
};
// Remove last added resolved user from the array and updates the hidden field control with a JSON string
PeoplePicker.prototype.PopResolvedUser = function () {
this._ResolvedUsers.pop();
this.PeoplePickerData.val(JSON.stringify(this._ResolvedUsers));
};
// Remove resolved user from the array and updates the hidden field control with a JSON string
PeoplePicker.prototype.RemoveResolvedUser = function (lookupValue) {
var newResolvedUsers = [];
for (var i = 0; i < this._ResolvedUsers.length; i++) {
var resolvedLookupValue = this._ResolvedUsers[i].Login ? this._ResolvedUsers[i].Login : this._ResolvedUsers[i].LookupId;
if (resolvedLookupValue != lookupValue) {
newResolvedUsers.push(this._ResolvedUsers[i]);
}
}
this._ResolvedUsers = newResolvedUsers;
this.PeoplePickerData.val(JSON.stringify(this._ResolvedUsers));
};
// Update the people picker control to show the newly added user
PeoplePicker.prototype.RecipientSelected = function (login, name, email) {
this.HideSelectionBox();
// Push new resolved user to list
this.PushResolvedUser(this.ResolvedUser(login, name, email));
// Update the resolved user display
this.PeoplePickerControl.html(this.ResolvedUsersToHtml());
// Prepare the edit control for a second user selection
this.PeoplePickerEdit.val('');
this.PeoplePickerEdit.focus();
this.OnSelectionChanged();
};
// Delete a resolved user
PeoplePicker.prototype.DeleteProcessedUser = function (lookupValue) {
this.RemoveResolvedUser(lookupValue);
this.PeoplePickerControl.html(this.ResolvedUsersToHtml());
this.PeoplePickerEdit.focus();
this.OnSelectionChanged();
};
// Function called when something went wrong with the user query (clientPeoplePickerSearchUser)
PeoplePicker.prototype.QueryFailure = function (queryNumber) {
alert('Error performing user search');
};
// Function called then the clientPeoplePickerSearchUser succeeded
PeoplePicker.prototype.QuerySuccess = function (queryNumber, searchResult) {
var results = this.SharePointContext.parseObjectFromJsonString(searchResult.get_value());
var txtResults = '';
var baseDisplayTemplate = '<div class=\'ms-bgHoverable\' style=\'width: 400px; padding: 4px;\' ng-click=\'{0}.RecipientSelected(\"{1}\", \"{2}\", \"{3}\")\'>{4}';
var displayTemplate = '';
if (this.ShowLoginName && this.ShowTitle) {
displayTemplate = baseDisplayTemplate + ' ({5})<br/>{6}</div>';
} else if (this.ShowLoginName || this.ShowTitle) {
displayTemplate = baseDisplayTemplate + ' ({6})</div>';
} else {
displayTemplate = baseDisplayTemplate + '</div>';
}
if (results) {
if (results.length > 0) {
// if this function is not the callback from the last issued query then just ignore it. This is needed to ensure a matching between
// what the user entered and what is shown in the query feedback window
if (queryNumber < this._lastQueryID) {
return;
}
var displayCount = results.length;
if (displayCount > this.MaxEntriesShown) {
displayCount = this.MaxEntriesShown;
}
for (var i = 0; i < displayCount; i++) {
var item = results[i];
var loginName = item['Key'];
var displayName = item['DisplayText'];
var title = item['EntityData']['Title'];
var email = item['EntityData']['Email'];
var loginNameDisplay = email;
if (loginName && loginName.indexOf('|') > -1) {
var segs = loginName.split('|');
loginNameDisplay = loginNameDisplay + " " + segs[segs.length - 1];
loginNameDisplay = loginNameDisplay.trim();
}
txtResults += this.Format(displayTemplate, this.InstanceName, loginName.replace("\\", "\\\\"), this.HtmlEncode(displayName), email, displayName, loginNameDisplay, title);
}
var resultDisplay = '';
txtResults += '<div class=\'ms-emphasisBorder\' style=\'width: 400px; padding: 4px; border-left: none; border-bottom: none; border-right: none; cursor: default;\'>';
if (results.length == 1) {
resultDisplay = 'Showing {0} result';
if (typeof resultsSingle != 'undefined') {
resultDisplay = resultsSingle;
}
txtResults += this.Format(resultDisplay, results.length) + '</div>';
} else if (displayCount != results.length) {
resultDisplay = "Showing {0} of {1} results. <B>Please refine further<B/>";
if (typeof resultsTooMany != 'undefined') {
resultDisplay = resultsTooMany;
}
txtResults += this.Format(resultDisplay, displayCount, results.length) + '</div>';
} else {
resultDisplay = "Showing {0} results";
if (typeof resultsMany != 'undefined') {
resultDisplay = resultsMany;
}
txtResults += this.Format(resultDisplay, results.length) + '</div>';
}
this.PeoplePickerDisplay.html(this.$compile(txtResults)(this.$scope));
//display the suggestion box
this.ShowSelectionBox();
}
else {
var searchbusy = '<div class=\'ms-emphasisBorder\' style=\'width: 400px; padding: 4px; border-left: none; border-bottom: none; border-right: none; cursor: default;\'>No results found</div>';
this.PeoplePickerDisplay.html(this.$compile(searchbusy)(this.$scope));
//display the suggestion box
this.ShowSelectionBox();
}
}
else {
//hide the suggestion box since results are null
this.HideSelectionBox();
}
};
// Initialize
PeoplePicker.prototype.Initialize = function () {
var scriptUrl = "";
var scriptRevision = "";
$('script').each(function (i, el) {
if (el.src.toLowerCase().indexOf('peoplepickercontrol.js') > -1) {
scriptUrl = el.src;
scriptRevision = scriptUrl.substring(scriptUrl.indexOf('.js') + 3);
scriptUrl = scriptUrl.substring(0, scriptUrl.indexOf('.js'));
}
});
// Load translation files
var resourcesFile = scriptUrl + "_resources." + this.Language.substring(0, 2).toLowerCase() + ".js";
if (scriptRevision.length > 0) {
resourcesFile += scriptRevision;
}
this.LoadScript(resourcesFile, function () {
});
// is there data in the hidden control...if so show it
if (this.PeoplePickerData.val().length > 0) {
// Deserialize JSON string into list of resolved users
this._ResolvedUsers = JSON.parse(this.PeoplePickerData.val());
// update the display of resolved users
this.PeoplePickerControl.html(this.ResolvedUsersToHtml());
}
//Capture reference to current control so that it can be used in event handlers
var parent = this;
//Capture click on parent DIV and set focus to the input control
this.PeoplePickerControl.parent().click(function (e) {
parent.PeoplePickerEdit.focus();
});
this.PeoplePickerEdit.keydown(function (event) {
var keynum = event.which;
//backspace
if (keynum == 8) {
//hide the suggestion box when backspace has been pressed
parent.HideSelectionBox();
// do we have text entered
var unvalidatedText = parent.PeoplePickerEdit.val();
if (unvalidatedText.length > 0) {
// delete the last entered character...meaning do nothing as this delete will happen as part of the keypress
}
else {
// are there resolved users, if not there's nothing to delete
if (parent._ResolvedUsers.length > 0) {
// remove the last added user
parent.PopResolvedUser();
// update the display
parent.PeoplePickerControl.html(parent.ResolvedUsersToHtml());
// focus back to input control
parent.PeoplePickerEdit.focus();
// Eat the backspace key
return false;
}
}
}
// An ascii character or a space has been pressed
else if (keynum >= 48 && keynum <= 90 || keynum == 32) {
// get the text entered before the keypress processing (so the last entered key is missing here)
var txt = parent.PeoplePickerEdit.val();
// keynum is not taking in account shift key and always results inthe uppercase value
if (event.shiftKey == false && keynum >= 65 && keynum <= 90) {
keynum += 32;
}
// Append the last entered character: since we're handling a keydown event this character has not yet been added hence the returned value misses the last character
txt += String.fromCharCode(keynum);
// we should have at least 1 character
if (txt.length > 0) {
var searchText = txt;
//ensure that MinimalCharactersBeforeSearching >= 1
if (parent.GetMinimalCharactersBeforeSearching() < 1) {
parent.SetMinimalCharactersBeforeSearching(1);
}
// only perform a query when we at least have two chars and we do not have a query running already
if (searchText.length >= parent.GetMinimalCharactersBeforeSearching()) {
resultDisplay = 'Searching...';
if (typeof resultsSearching != 'undefined') {
resultDisplay = resultsSearching;
}
var searchbusy = parent.Format('<div class=\'ms-emphasisBorder\' style=\'width: 400px; padding: 4px; border-left: none; border-bottom: none; border-right: none; cursor: default;\'>{0}</div>', resultDisplay);
parent.PeoplePickerDisplay.html(parent.$compile(searchbusy)(parent.$scope));
//display the suggestion box
parent.ShowSelectionBox();
var query = new SP.UI.ApplicationPages.ClientPeoplePickerQueryParameters();
query.set_allowMultipleEntities(false);
query.set_maximumEntitySuggestions(2000);
query.set_principalType(parent.GetPrincipalType());
query.set_principalSource(15);
query.set_queryString(searchText);
var searchResult = SP.UI.ApplicationPages.ClientPeoplePickerWebServiceInterface.clientPeoplePickerSearchUser(parent.SharePointContext, query);
// update the global queryID variable so that we can correlate incoming delegate calls later on
parent._queryID = parent._queryID + 1;
var queryIDToPass = parent._queryID;
parent._lastQueryID = queryIDToPass;
// make the SharePoint request
parent.SharePointContext.executeQueryAsync(Function.createDelegate(this, function () { parent.QuerySuccess(queryIDToPass, searchResult); }),
Function.createDelegate(this, function () { parent.QueryFailure(queryIDToPass); }));
}
}
}
//tab or escape
else if (keynum == 9 || keynum == 27) {
//hide the suggestion box
parent.HideSelectionBox();
}
});
};
return PeoplePicker;
})();
CAMControl.PeoplePicker = PeoplePicker;
})(CAMControl || (CAMControl = {}));
})();
| {
"content_hash": "73683ec9c5f2c1c78cccf5065abc8c74",
"timestamp": "",
"source": "github",
"line_count": 566,
"max_line_length": 251,
"avg_line_length": 52.13250883392226,
"alnum_prop": 0.5076083641169892,
"repo_name": "jasonvenema/sharepoint-angular-peoplepicker",
"id": "fa0f93524c508a843c1307db18f335b0fe46cb1b",
"size": "29509",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "sp-peoplepicker.js",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "CSS",
"bytes": "1245"
},
{
"name": "JavaScript",
"bytes": "28285"
}
],
"symlink_target": ""
} |
package io.strimzi.crdgenerator.annotations;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
/**
* Annotation for classes which represent a choice between two or more possibilities identified by the
* {@link Alternative}-annotated properties of the annotated class.
* The JSON schema generated uses a {@code oneOf} constraint to force the choice between the alternative properties.
* The annotated class will typically need to have custom Jackson serialization which can determine from the serialized
* form which of the alternatives to deserialize.
*/
@Retention(RetentionPolicy.RUNTIME)
@Target({ElementType.TYPE})
public @interface Alternation {
}
| {
"content_hash": "5d19d7169d50ce044de2bccc57f7f09a",
"timestamp": "",
"source": "github",
"line_count": 19,
"max_line_length": 119,
"avg_line_length": 40.68421052631579,
"alnum_prop": 0.8072445019404916,
"repo_name": "ppatierno/kaas",
"id": "e0b061f545623621f544cf1f9201f9c67b77f88d",
"size": "911",
"binary": false,
"copies": "2",
"ref": "refs/heads/main",
"path": "crd-generator/src/main/java/io/strimzi/crdgenerator/annotations/Alternation.java",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "Python",
"bytes": "3237"
},
{
"name": "Shell",
"bytes": "6301"
}
],
"symlink_target": ""
} |
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<!-- NewPage -->
<html lang="en">
<head>
<!-- Generated by javadoc (version 1.7.0_25) on Thu Jan 30 08:25:22 GMT 2014 -->
<title>org.vertx.java.core.sockjs</title>
<meta name="date" content="2014-01-30">
<link rel="stylesheet" type="text/css" href="../../../../../stylesheet.css" title="Style">
</head>
<body>
<h1 class="bar"><a href="../../../../../org/vertx/java/core/sockjs/package-summary.html" target="classFrame">org.vertx.java.core.sockjs</a></h1>
<div class="indexContainer">
<h2 title="Interfaces">Interfaces</h2>
<ul title="Interfaces">
<li><a href="EventBusBridgeHook.html" title="interface in org.vertx.java.core.sockjs" target="classFrame"><i>EventBusBridgeHook</i></a></li>
<li><a href="SockJSServer.html" title="interface in org.vertx.java.core.sockjs" target="classFrame"><i>SockJSServer</i></a></li>
<li><a href="SockJSSocket.html" title="interface in org.vertx.java.core.sockjs" target="classFrame"><i>SockJSSocket</i></a></li>
</ul>
<h2 title="Classes">Classes</h2>
<ul title="Classes">
<li><a href="EventBusBridge.html" title="class in org.vertx.java.core.sockjs" target="classFrame">EventBusBridge</a></li>
</ul>
</div>
</body>
</html>
| {
"content_hash": "304d4296ca9a9ea51f8c787d0467e884",
"timestamp": "",
"source": "github",
"line_count": 25,
"max_line_length": 144,
"avg_line_length": 50.4,
"alnum_prop": 0.6896825396825397,
"repo_name": "fhg-fokus-nubomedia/signaling-plane",
"id": "1bb95489beb49b2dd12742ed49a875de013b8bb1",
"size": "1260",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "vertx/api-docs/java/api/org/vertx/java/core/sockjs/package-frame.html",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "CSS",
"bytes": "12152"
},
{
"name": "Groff",
"bytes": "22"
},
{
"name": "HTML",
"bytes": "2637100"
},
{
"name": "Java",
"bytes": "5622899"
},
{
"name": "JavaScript",
"bytes": "3448641"
},
{
"name": "Python",
"bytes": "161709"
},
{
"name": "Shell",
"bytes": "8658"
}
],
"symlink_target": ""
} |
var configuration = require('./configuration'),
db = require('./db'),
google = require('./google'),
Promise = require('bluebird'),
uuid = require('node-uuid');
/**
* @param {string} email
* @return {!Promise<!google.auth.JWT>}
*/
function authenticate (email) {
return google.authenticate({
email: email,
scopes: ['https://www.googleapis.com/auth/drive.readonly'],
serviceAccount: {
email: configuration.GOOGLE_SERVICE_ACCOUNT_EMAIL,
privateKey: configuration.GOOGLE_SERVICE_ACCOUNT_PRIVATE_KEY
}
});
}
/**
* @param {!Object} user
* @return {!Promise}
*/
function createChannel (user) {
console.log('Creating channel for ' + user.email);
return watchChanges()
.then(storeChannel);
/**
* @param {!Object} watchResponse
* @return {!Promise}
*/
function storeChannel (watchResponse) {
return db.channels.create({
expires: watchResponse.expiration,
id: watchResponse.id,
resourceId: watchResponse.resourceId,
userId: user.id
});
}
/**
* @return {!Promise<!Object>}
*/
function watchChanges () {
return authenticate(user.email).then(function (auth) {
return google.drive.changes.watch({
address: configuration.NOTIFICATION_URL,
auth: auth,
channelId: uuid.v4()
});
});
}
}
/**
* @return {!Promise}
*/
function createNewChannels () {
return db.users.listWithoutChannel()
.map(createChannel);
}
/**
* @param {!Object} channel
* @return {!Promise}
*/
function recreateChannel (channel) {
return db.users.get(channel.user_id).then(function (user) {
console.log('Recreating channel for ' + user.email);
return createChannel(user)
.then(removeChannel.bind(null, channel, user));
});
}
/**
* @return {!Promise}
*/
function recreateExpiringChannels () {
var ONE_DAY = 1000 * 60 * 60 * 24;
return db.channels.listExpiringIn(ONE_DAY)
.map(recreateChannel);
}
/**
* @param {!Object} channel
* @param {!Object} user
* @return {!Promise}
*/
function removeChannel (channel, user) {
return stopChannel()
.catch(function () {})
.then(dropChannel);
/**
* @return {!Promise}
*/
function dropChannel () {
return db.channels.remove([channel.id]);
}
/**
* @return {!Promise}
*/
function stopChannel () {
return authenticate(user.email).then(function (auth) {
return google.drive.channels.stop({
auth: auth,
id: channel.id,
resourceId: channel.resource_id
});
});
}
}
/**
* @return {!Promise}
*/
function refresh () {
return db.channels.removeDangling()
.then(recreateExpiringChannels)
.then(createNewChannels);
}
/**
* @return {!Promise}
*/
function removeAll () {
return db.channels.list().then(function (channels) {
return getUserMap().then(function (userMap) {
var index = 0;
return (function removeNextChannel () {
console.log('Removing channel ' + (index + 1) + '/' + channels.length);
var channel = channels[index];
var user = userMap[channel.user_id];
return removeChannel(channel, user).then(function () {
index++;
return removeNextChannel();
});
}(0));
});
});
/**
* @return {!Promise<!Object<!Object>>}
*/
function getUserMap() {
return db.users.list().then(function (users) {
return users.reduce(function (userMap, user) {
userMap[user.id] = user;
return userMap;
}, {});
});
}
}
module.exports = {
refresh: refresh,
removeAll: removeAll
};
| {
"content_hash": "a935e133b72ce0938281e44715b30c85",
"timestamp": "",
"source": "github",
"line_count": 163,
"max_line_length": 79,
"avg_line_length": 22.11042944785276,
"alnum_prop": 0.607103218645949,
"repo_name": "chrisdoble/drive-hipchat",
"id": "c759d868dd1afb8763c5aacaec32d358dedfef95",
"size": "3604",
"binary": false,
"copies": "2",
"ref": "refs/heads/master",
"path": "src/channels.js",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "JavaScript",
"bytes": "25091"
}
],
"symlink_target": ""
} |
<?php
namespace Jhg\ExpertSystem\Knowledge;
use Jhg\ExpertSystem\Knowledge\Exception\KnowledgeLoaderError;
use Jhg\ExpertSystem\KnowledgeBase\KnowledgeLoaderInterface;
/**
* Class KnowledgeJsonLoader
*
* @package Jhg\ExpertSystem\Knowledge
*/
class KnowledgeJsonLoader implements KnowledgeLoaderInterface
{
/**
* @var array
*/
protected $data;
/**
* @param string $jsonPath
*
* @throws KnowledgeLoaderError
*/
public function __construct($jsonPath)
{
if (!file_exists($jsonPath)) {
throw new KnowledgeLoaderError(sprintf('Json file does not exist at "%s".', $jsonPath));
}
$jsonString = file_get_contents($jsonPath);
$data = json_decode($jsonString, true);
if (!$data) {
throw new KnowledgeLoaderError(sprintf('Can not decode json.', $jsonPath));
}
$this->data = $data;
}
/**
* @param KnowledgeBase $knowledgeBase
*/
public function load(KnowledgeBase $knowledgeBase)
{
foreach ($this->data['facts'] as $name => $value) {
$knowledgeBase->add(Fact::factory($name, $value));
}
foreach ($this->data['rules'] as $condition => $data) {
if (is_string($data)) {
$action = $data;
$priority = 0;
} else {
$action = $data['action'];
$priority = isset($data['priority']) ? $data['priority'] : 0;
}
$knowledgeBase->add(Rule::factory($condition, $action, $priority));
}
}
}
| {
"content_hash": "81cc0075f94e024fcc9c6dbc3ceaa3e9",
"timestamp": "",
"source": "github",
"line_count": 66,
"max_line_length": 100,
"avg_line_length": 24.166666666666668,
"alnum_prop": 0.5636363636363636,
"repo_name": "blackmore466/expert-system",
"id": "55a6f4c5b09cb43156b176afbcf1fc2de9d39b98",
"size": "1836",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "InferenceEngine/Knowledge/KnowledgeJsonLoader.php",
"mode": "33188",
"license": "bsd-3-clause",
"language": [
{
"name": "ApacheConf",
"bytes": "284"
},
{
"name": "Batchfile",
"bytes": "1080"
},
{
"name": "CSS",
"bytes": "1733"
},
{
"name": "PHP",
"bytes": "1294702"
}
],
"symlink_target": ""
} |
Oops.. something went wrong.
| {
"content_hash": "38eafc5f769b52c0fd5872527dbd48c8",
"timestamp": "",
"source": "github",
"line_count": 1,
"max_line_length": 28,
"avg_line_length": 29,
"alnum_prop": 0.7586206896551724,
"repo_name": "mkirvela/kyltti.com",
"id": "2b06f84905a55f92002ff82d81c5c726c46c1a66",
"size": "29",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "webfront/templates/500.html",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "JavaScript",
"bytes": "7447"
},
{
"name": "Python",
"bytes": "14260"
}
],
"symlink_target": ""
} |
FN="yeastCC_1.28.0.tar.gz"
URLS=(
"https://bioconductor.org/packages/3.11/data/experiment/src/contrib/yeastCC_1.28.0.tar.gz"
"https://bioarchive.galaxyproject.org/yeastCC_1.28.0.tar.gz"
"https://depot.galaxyproject.org/software/bioconductor-yeastcc/bioconductor-yeastcc_1.28.0_src_all.tar.gz"
)
MD5="5097e470afb2db311c66a1cd3a62ff36"
# Use a staging area in the conda dir rather than temp dirs, both to avoid
# permission issues as well as to have things downloaded in a predictable
# manner.
STAGING=$PREFIX/share/$PKG_NAME-$PKG_VERSION-$PKG_BUILDNUM
mkdir -p $STAGING
TARBALL=$STAGING/$FN
SUCCESS=0
for URL in ${URLS[@]}; do
curl $URL > $TARBALL
[[ $? == 0 ]] || continue
# Platform-specific md5sum checks.
if [[ $(uname -s) == "Linux" ]]; then
if md5sum -c <<<"$MD5 $TARBALL"; then
SUCCESS=1
break
fi
else if [[ $(uname -s) == "Darwin" ]]; then
if [[ $(md5 $TARBALL | cut -f4 -d " ") == "$MD5" ]]; then
SUCCESS=1
break
fi
fi
fi
done
if [[ $SUCCESS != 1 ]]; then
echo "ERROR: post-link.sh was unable to download any of the following URLs with the md5sum $MD5:"
printf '%s\n' "${URLS[@]}"
exit 1
fi
# Install and clean up
R CMD INSTALL --library=$PREFIX/lib/R/library $TARBALL
rm $TARBALL
rmdir $STAGING
| {
"content_hash": "ea97ba28fcfaf45b5d2d525882232d41",
"timestamp": "",
"source": "github",
"line_count": 45,
"max_line_length": 108,
"avg_line_length": 28.333333333333332,
"alnum_prop": 0.6635294117647059,
"repo_name": "roryk/recipes",
"id": "d42507233dad32900f516d60822d1d11c69a75d8",
"size": "1287",
"binary": false,
"copies": "3",
"ref": "refs/heads/master",
"path": "recipes/bioconductor-yeastcc/post-link.sh",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "Batchfile",
"bytes": "41916"
},
{
"name": "Makefile",
"bytes": "539"
},
{
"name": "Perl",
"bytes": "133"
},
{
"name": "Python",
"bytes": "38996"
},
{
"name": "Shell",
"bytes": "229850"
}
],
"symlink_target": ""
} |
mkdir -p NEW
mkdir -p DIFF
passed=0
failed=0
cat /dev/null > failure-outputs.txt
runComplexTests()
{
for i in *.sh
do
case $i in TEST*.sh) continue;; esac
if sh ./$i
then
passed=`expr $passed + 1`
else
failed=`expr $failed + 1`
fi
done
}
runSimpleTests()
{
only=$1
echo $passed >.passed
echo $failed >.failed
cat TESTLIST | while read name input output options
do
case $name in
\#*) continue;;
'') continue;;
esac
[ "$only" != "" -a "$name" != "$only" ] && continue
if ./TESTonce $name $input $output "$options"
then
passed=`expr $passed + 1`
echo $passed >.passed
else
failed=`expr $failed + 1`
echo $failed >.failed
fi
[ "$only" != "" -a "$name" = "$only" ] && break
done
# I hate shells with their stupid, useless subshells.
passed=`cat .passed`
failed=`cat .failed`
}
if [ $# -eq 0 ]
then
runComplexTests
runSimpleTests
elif [ $# -eq 1 ]
then
runSimpleTests $1
else
echo "Usage: $0 [test_name]"
exit 30
fi
# exit with number of failing tests.
echo '------------------------------------------------'
printf "%4u tests failed\n" $failed
printf "%4u tests passed\n" $passed
echo
echo
cat failure-outputs.txt
echo
echo
exit $failed
| {
"content_hash": "603807f6dd95f6b9ef6f49f2a76a3991",
"timestamp": "",
"source": "github",
"line_count": 69,
"max_line_length": 55,
"avg_line_length": 18.405797101449274,
"alnum_prop": 0.5787401574803149,
"repo_name": "unix1986/universe",
"id": "924e5f5e53b37b864f36cc015d8988a2d08f1ca6",
"size": "1281",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "tool/tcpdump-4.6.2/tests/TESTrun.sh",
"mode": "33261",
"license": "bsd-2-clause",
"language": [
{
"name": "Assembly",
"bytes": "532"
},
{
"name": "Awk",
"bytes": "4122"
},
{
"name": "Bison",
"bytes": "67882"
},
{
"name": "C",
"bytes": "12970053"
},
{
"name": "C++",
"bytes": "9620178"
},
{
"name": "CMake",
"bytes": "3479525"
},
{
"name": "CSS",
"bytes": "3624"
},
{
"name": "DTrace",
"bytes": "10988"
},
{
"name": "Emacs Lisp",
"bytes": "14206"
},
{
"name": "FORTRAN",
"bytes": "5057"
},
{
"name": "Groff",
"bytes": "311977"
},
{
"name": "HTML",
"bytes": "438654"
},
{
"name": "Java",
"bytes": "694123"
},
{
"name": "Lua",
"bytes": "11896"
},
{
"name": "M",
"bytes": "5971"
},
{
"name": "Makefile",
"bytes": "205335"
},
{
"name": "Objective-C",
"bytes": "38700"
},
{
"name": "Objective-C++",
"bytes": "607"
},
{
"name": "PHP",
"bytes": "1498"
},
{
"name": "Perl",
"bytes": "333017"
},
{
"name": "Pike",
"bytes": "1338"
},
{
"name": "Python",
"bytes": "406099"
},
{
"name": "Rebol",
"bytes": "291"
},
{
"name": "Ruby",
"bytes": "9647"
},
{
"name": "Scala",
"bytes": "1704222"
},
{
"name": "Scilab",
"bytes": "120244"
},
{
"name": "Shell",
"bytes": "313916"
},
{
"name": "Smarty",
"bytes": "1023"
},
{
"name": "Tcl",
"bytes": "365772"
},
{
"name": "TeX",
"bytes": "23"
},
{
"name": "VimL",
"bytes": "13304"
},
{
"name": "XSLT",
"bytes": "18239"
}
],
"symlink_target": ""
} |
using System.Collections.Generic;
namespace Affecto.AuditTrail.WebApi.Model
{
public class AuditTrailResult
{
public List<AuditTrailEntry> Items { get; set; }
public long ResultCount { get; set; }
public AuditTrailResult()
{
Items = new List<AuditTrailEntry>();
}
}
} | {
"content_hash": "07535a369389aa5a6e3baf6c3772c1d7",
"timestamp": "",
"source": "github",
"line_count": 15,
"max_line_length": 56,
"avg_line_length": 22.266666666666666,
"alnum_prop": 0.6137724550898204,
"repo_name": "affecto/dotnet-AuditTrail",
"id": "bb6f3079dd4f0b0a1ab38173638a713c8c507b54",
"size": "336",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "AuditTrail.WebApi/Model/AuditTrailResult.cs",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "C#",
"bytes": "69458"
}
],
"symlink_target": ""
} |
//********************************************************************
//*
//* Warning: This file was generated by ecore4CPP Generator
//*
//********************************************************************
#ifndef UML_ACTIVITYPARAMETERNODEACTIVITYPARAMETERNODEIMPL_HPP
#define UML_ACTIVITYPARAMETERNODEACTIVITYPARAMETERNODEIMPL_HPP
//*********************************
// generated Includes
// namespace macro header include
#include "uml/uml.hpp"
// model includes
#include "../ActivityParameterNode.hpp"
#include "uml/impl/ObjectNodeImpl.hpp"
//*********************************
namespace uml
{
class UML_API ActivityParameterNodeImpl : virtual public ObjectNodeImpl, virtual public ActivityParameterNode
{
public:
ActivityParameterNodeImpl(const ActivityParameterNodeImpl & obj);
virtual std::shared_ptr<ecore::EObject> copy() const;
ActivityParameterNodeImpl& operator=(ActivityParameterNodeImpl const&);
protected:
friend class umlFactoryImpl;
ActivityParameterNodeImpl();
virtual std::shared_ptr<uml::ActivityParameterNode> getThisActivityParameterNodePtr() const;
virtual void setThisActivityParameterNodePtr(std::weak_ptr<uml::ActivityParameterNode> thisActivityParameterNodePtr);
//Additional constructors for the containments back reference
ActivityParameterNodeImpl(std::weak_ptr<uml::Activity> par_activity);
//Additional constructors for the containments back reference
ActivityParameterNodeImpl(std::weak_ptr<uml::StructuredActivityNode> par_inStructuredNode);
//Additional constructors for the containments back reference
ActivityParameterNodeImpl(std::weak_ptr<uml::Namespace> par_namespace);
//Additional constructors for the containments back reference
ActivityParameterNodeImpl(std::weak_ptr<uml::Element> par_owner);
public:
//destructor
virtual ~ActivityParameterNodeImpl();
//*********************************
// Operations
//*********************************
/*!
The parameter of an ActivityParameterNode must be from the containing Activity.
activity.ownedParameter->includes(parameter)
*/
virtual bool has_parameters(Any diagnostics,std::shared_ptr<std::map < Any, Any>> context) ;
/*!
An ActivityParameterNode may have all incoming ActivityEdges or all outgoing ActivityEdges, but it must not have both incoming and outgoing ActivityEdges.
incoming->isEmpty() or outgoing->isEmpty()
*/
virtual bool no_edges(Any diagnostics,std::shared_ptr<std::map < Any, Any>> context) ;
/*!
An ActivityParameterNode with no incoming ActivityEdges and one or more outgoing ActivityEdges must have a parameter with direction in or inout.
(outgoing->notEmpty() and incoming->isEmpty()) implies
(parameter.direction = ParameterDirectionKind::_'in' or
parameter.direction = ParameterDirectionKind::inout)
*/
virtual bool no_incoming_edges(Any diagnostics,std::shared_ptr<std::map < Any, Any>> context) ;
/*!
An ActivityParameterNode with no outgoing ActivityEdges and one or more incoming ActivityEdges must have a parameter with direction out, inout, or return.
(incoming->notEmpty() and outgoing->isEmpty()) implies
(parameter.direction = ParameterDirectionKind::out or
parameter.direction = ParameterDirectionKind::inout or
parameter.direction = ParameterDirectionKind::return)
*/
virtual bool no_outgoing_edges(Any diagnostics,std::shared_ptr<std::map < Any, Any>> context) ;
/*!
The type of an ActivityParameterNode is the same as the type of its parameter.
type = parameter.type
*/
virtual bool same_type(Any diagnostics,std::shared_ptr<std::map < Any, Any>> context) ;
//*********************************
// Attribute Getters & Setters
//*********************************
//*********************************
// Reference Getters & Setters
//*********************************
/*!
The Parameter for which the ActivityParameterNode will be accepting or providing values.
<p>From package UML::Activities.</p>
*/
virtual std::shared_ptr<uml::Parameter> getParameter() const ;
/*!
The Parameter for which the ActivityParameterNode will be accepting or providing values.
<p>From package UML::Activities.</p>
*/
virtual void setParameter(std::shared_ptr<uml::Parameter>) ;
//*********************************
// Union Reference Getters
//*********************************
/*!
ActivityGroups containing the ActivityNode.
<p>From package UML::Activities.</p>
*/
virtual std::shared_ptr<Union<uml::ActivityGroup>> getInGroup() const ;
/*!
The Elements owned by this Element.
<p>From package UML::CommonStructure.</p>
*/
virtual std::shared_ptr<Union<uml::Element>> getOwnedElement() const ;
/*!
The Element that owns this Element.
<p>From package UML::CommonStructure.</p>
*/
virtual std::weak_ptr<uml::Element> getOwner() const ;
/*!
The RedefinableElement that is being redefined by this element.
<p>From package UML::Classification.</p>
*/
virtual std::shared_ptr<Union<uml::RedefinableElement>> getRedefinedElement() const ;
//*********************************
// Container Getter
//*********************************
virtual std::shared_ptr<ecore::EObject> eContainer() const ;
//*********************************
// Persistence Functions
//*********************************
virtual void load(std::shared_ptr<persistence::interfaces::XLoadHandler> loadHandler) ;
virtual void loadAttributes(std::shared_ptr<persistence::interfaces::XLoadHandler> loadHandler, std::map<std::string, std::string> attr_list);
virtual void loadNode(std::string nodeName, std::shared_ptr<persistence::interfaces::XLoadHandler> loadHandler);
virtual void resolveReferences(const int featureID, std::vector<std::shared_ptr<ecore::EObject> > references) ;
virtual void save(std::shared_ptr<persistence::interfaces::XSaveHandler> saveHandler) const ;
virtual void saveContent(std::shared_ptr<persistence::interfaces::XSaveHandler> saveHandler) const;
protected:
virtual std::shared_ptr<ecore::EClass> eStaticClass() const;
//*********************************
// EStructuralFeature Get/Set/IsSet
//*********************************
virtual Any eGet(int featureID, bool resolve, bool coreType) const ;
virtual bool eSet(int featureID, Any newValue) ;
virtual bool internalEIsSet(int featureID) const ;
//*********************************
// EOperation Invoke
//*********************************
virtual Any eInvoke(int operationID, std::shared_ptr<std::list<Any>> arguments) ;
private:
std::weak_ptr<uml::ActivityParameterNode> m_thisActivityParameterNodePtr;
};
}
#endif /* end of include guard: UML_ACTIVITYPARAMETERNODEACTIVITYPARAMETERNODEIMPL_HPP */
| {
"content_hash": "d8118a9cbc8c2582548c6361946ba6f1",
"timestamp": "",
"source": "github",
"line_count": 171,
"max_line_length": 157,
"avg_line_length": 40.35672514619883,
"alnum_prop": 0.6497609042167801,
"repo_name": "MDE4CPP/MDE4CPP",
"id": "b344b9ccaa01ebb3ec8ca7e4cb9e2ae5afb49ba1",
"size": "6901",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "src/uml/uml/src_gen/uml/impl/ActivityParameterNodeImpl.hpp",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "ANTLR",
"bytes": "22599"
},
{
"name": "Batchfile",
"bytes": "25126"
},
{
"name": "C++",
"bytes": "45031588"
},
{
"name": "CMake",
"bytes": "127756"
},
{
"name": "Java",
"bytes": "196231"
},
{
"name": "Shell",
"bytes": "3055"
}
],
"symlink_target": ""
} |
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Web.Script.Serialization;
using Gherkin.Ast;
namespace Gherkin
{
public interface IGherkinDialectProvider
{
GherkinDialect DefaultDialect { get; }
GherkinDialect GetDialect(string language, Location location);
}
public class GherkinDialectProvider : IGherkinDialectProvider
{
protected class GherkinLanguageSetting
{
// ReSharper disable InconsistentNaming
public string name;
public string native;
public string[] feature;
public string[] background;
public string[] scenario;
public string[] scenarioOutline;
public string[] examples;
public string[] given;
public string[] when;
public string[] then;
public string[] and;
public string[] but;
public string[] asA;
public string[] iWant;
public string[] soThat;
public string[] qualityAttribute;
public string[] scenarioContribution;
public string[] whichMayImpact;
// ReSharper restore InconsistentNaming
}
private readonly Lazy<GherkinDialect> defaultDialect;
public GherkinDialect DefaultDialect
{
get { return defaultDialect.Value; }
}
public GherkinDialectProvider(string defaultLanguage = "en")
{
defaultDialect = new Lazy<GherkinDialect>(() => GetDialect(defaultLanguage, null));
}
public virtual GherkinDialect GetDialect(string language, Location location)
{
var gherkinLanguageSettings = LoadLanguageSettings();
return GetDialect(language, gherkinLanguageSettings, location);
}
protected virtual Dictionary<string, GherkinLanguageSetting> LoadLanguageSettings()
{
const string languageFileName = "gherkin-languages.json";
var resourceStream = typeof(GherkinDialectProvider).Assembly.GetManifestResourceStream(typeof(GherkinDialectProvider), languageFileName);
if (resourceStream == null)
throw new InvalidOperationException("Gherkin language resource not found: " + languageFileName);
var languagesFileContent = new StreamReader(resourceStream).ReadToEnd();
return ParseJsonContent(languagesFileContent);
}
protected Dictionary<string, GherkinLanguageSetting> ParseJsonContent(string languagesFileContent)
{
var jsonSerializer = new JavaScriptSerializer();
return jsonSerializer.Deserialize<Dictionary<string, GherkinLanguageSetting>>(languagesFileContent);
}
protected virtual GherkinDialect GetDialect(string language, Dictionary<string, GherkinLanguageSetting> gherkinLanguageSettings, Location location)
{
GherkinLanguageSetting languageSettings;
if (!gherkinLanguageSettings.TryGetValue(language, out languageSettings))
throw new NoSuchLanguageException(language, location);
return CreateGherkinDialect(language, languageSettings);
}
protected GherkinDialect CreateGherkinDialect(string language, GherkinLanguageSetting languageSettings)
{
return new GherkinDialect(
language,
ParseTitleKeywords(languageSettings.feature),
ParseTitleKeywords(languageSettings.background),
ParseTitleKeywords(languageSettings.scenario),
ParseTitleKeywords(languageSettings.scenarioOutline),
ParseTitleKeywords(languageSettings.examples),
ParseStepKeywords(languageSettings.given),
ParseStepKeywords(languageSettings.when),
ParseStepKeywords(languageSettings.then),
ParseStepKeywords(languageSettings.and),
ParseStepKeywords(languageSettings.but),
languageSettings.asA,
languageSettings.iWant,
languageSettings.soThat,
languageSettings.qualityAttribute,
languageSettings.scenarioContribution,
languageSettings.whichMayImpact
);
}
private string[] ParseStepKeywords(string[] stepKeywords)
{
return stepKeywords;
}
private string[] ParseTitleKeywords(string[] keywords)
{
return keywords;
}
protected static GherkinDialect GetFactoryDefault()
{
return new GherkinDialect(
"en",
new[] {"Feature"},
new[] {"Background"},
new[] {"Scenario"},
new[] {"Scenario Outline", "Scenario Template"},
new[] {"Examples", "Scenarios"},
new[] {"* ", "Given "},
new[] {"* ", "When " },
new[] {"* ", "Then " },
new[] {"* ", "And " },
new[] {"* ", "But " },
new[] {"* ","As a "},
new[] {"* ","I want " },
new[] {"* ", "So that " },
new[] {"Without ignoring"},
new[] { "Breaking the ", "Which breaks ", "Contributing to break ",
"Helping the ", "Which helps ", "Contributing to help ",
"Hurting the ", "Which hurts ", "Contributing to hurt ",
"Making the ", "Which makes ", "Contributing to make ",
"With some positive contribution to ",
"With some negative contribution to "},
new string[] {"Which may impact "});
}
}
}
| {
"content_hash": "7b320efc0d1cf7635ab783bad7464644",
"timestamp": "",
"source": "github",
"line_count": 149,
"max_line_length": 155,
"avg_line_length": 40.2751677852349,
"alnum_prop": 0.5739043492751208,
"repo_name": "moreirap/gherkin3",
"id": "abe8b47bf58e2985c0e50ed989a43052cdd8af9b",
"size": "6003",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "dotnet/Gherkin/GherkinDialectProvider.cs",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "Batchfile",
"bytes": "1081"
},
{
"name": "C#",
"bytes": "387425"
},
{
"name": "Cucumber",
"bytes": "17947"
},
{
"name": "Go",
"bytes": "159375"
},
{
"name": "HTML",
"bytes": "261"
},
{
"name": "JSONiq",
"bytes": "1060"
},
{
"name": "Java",
"bytes": "151567"
},
{
"name": "JavaScript",
"bytes": "94374"
},
{
"name": "Makefile",
"bytes": "14672"
},
{
"name": "Python",
"bytes": "102957"
},
{
"name": "Ruby",
"bytes": "94613"
},
{
"name": "Shell",
"bytes": "1335"
}
],
"symlink_target": ""
} |
ProjectileObject::ProjectileObject(){}
ProjectileObject::~ProjectileObject(){}
void ProjectileObject::InitProjectile() {
setMatrix(IDENTITY_M4);
isDrawn = false;
lifeTime = 0.0f;
gravity = 9.8f;
speed = 0.3f;
}
bool ProjectileObject::getIsDrawn() { return isDrawn; }
matrix4 ProjectileObject::getMatrix() { return m_m4Projectile; }
float ProjectileObject::getLifeTime() { return lifeTime; }
void ProjectileObject::Draw(CameraManagerSingleton* m_pCameraMngr) {}
void ProjectileObject::setMatrix(matrix4 m) { m_m4Projectile = m; }
void ProjectileObject::setIsDrawn(bool drawn) { isDrawn = drawn; }
bool ProjectileObject::isColliding() { return false; }
void ProjectileObject::Draw(CameraManagerSingleton* m_pCameraMngr) {} | {
"content_hash": "e9a3d6fc5507168ab043425c84855eb9",
"timestamp": "",
"source": "github",
"line_count": 22,
"max_line_length": 69,
"avg_line_length": 33.18181818181818,
"alnum_prop": 0.7643835616438356,
"repo_name": "ntancredi/BIRB",
"id": "9ff364b8bb42d0caa8ac57aa6feccab8c5b6e129",
"size": "762",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "00_Sandbox/ProjectileObject.cpp",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "C",
"bytes": "1300196"
},
{
"name": "C++",
"bytes": "2745613"
},
{
"name": "CMake",
"bytes": "1566"
},
{
"name": "GLSL",
"bytes": "25362"
},
{
"name": "Objective-C",
"bytes": "11368"
}
],
"symlink_target": ""
} |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>coquelicot: Not compatible 👼</title>
<link rel="shortcut icon" type="image/png" href="../../../../../favicon.png" />
<link href="../../../../../bootstrap.min.css" rel="stylesheet">
<link href="../../../../../bootstrap-custom.css" rel="stylesheet">
<link href="//maxcdn.bootstrapcdn.com/font-awesome/4.2.0/css/font-awesome.min.css" rel="stylesheet">
<script src="../../../../../moment.min.js"></script>
<!-- HTML5 Shim and Respond.js IE8 support of HTML5 elements and media queries -->
<!-- WARNING: Respond.js doesn't work if you view the page via file:// -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/html5shiv/3.7.2/html5shiv.min.js"></script>
<script src="https://oss.maxcdn.com/respond/1.4.2/respond.min.js"></script>
<![endif]-->
</head>
<body>
<div class="container">
<div class="navbar navbar-default" role="navigation">
<div class="container-fluid">
<div class="navbar-header">
<a class="navbar-brand" href="../../../../.."><i class="fa fa-lg fa-flag-checkered"></i> Coq bench</a>
</div>
<div id="navbar" class="collapse navbar-collapse">
<ul class="nav navbar-nav">
<li><a href="../..">clean / released</a></li>
<li class="active"><a href="">8.8.2 / coquelicot - 3.0.3+8.11</a></li>
</ul>
</div>
</div>
</div>
<div class="article">
<div class="row">
<div class="col-md-12">
<a href="../..">« Up</a>
<h1>
coquelicot
<small>
3.0.3+8.11
<span class="label label-info">Not compatible 👼</span>
</small>
</h1>
<p>📅 <em><script>document.write(moment("2022-06-06 01:32:17 +0000", "YYYY-MM-DD HH:mm:ss Z").fromNow());</script> (2022-06-06 01:32:17 UTC)</em><p>
<h2>Context</h2>
<pre># Packages matching: installed
# Name # Installed # Synopsis
base-bigarray base
base-threads base
base-unix base
camlp5 7.14 Preprocessor-pretty-printer of OCaml
conf-findutils 1 Virtual package relying on findutils
conf-perl 2 Virtual package relying on perl
coq 8.8.2 Formal proof management system
num 1.4 The legacy Num library for arbitrary-precision integer and rational arithmetic
ocaml 4.09.1 The OCaml compiler (virtual package)
ocaml-base-compiler 4.09.1 Official release 4.09.1
ocaml-config 1 OCaml Switch Configuration
ocamlfind 1.9.3 A library manager for OCaml
# opam file:
opam-version: "2.0"
maintainer: "[email protected]"
homepage: "http://coquelicot.saclay.inria.fr/"
dev-repo: "git+https://gitlab.inria.fr/coquelicot/coquelicot.git"
bug-reports: "https://gitlab.inria.fr/coquelicot/coquelicot/issues"
license: "LGPL-3.0-or-later"
build: [
["./autogen.sh"]
["./configure"]
["./remake" "-j%{jobs}%"]
]
install: ["./remake" "install"]
depends: [
"coq" {= "8.11.0"}
"coq-mathcomp-ssreflect" {>= "1.6"}
("conf-g++" {build} | "conf-clang" {build})
"conf-autoconf" {build}
]
tags: [ "keyword:real analysis" "keyword:topology" "keyword:filters" "keyword:metric spaces" "category:Mathematics/Real Calculus and Topology" "date:2020-01-14" "logpath:Coquelicot"]
authors: [ "Sylvie Boldo <[email protected]>" "Catherine Lelay <[email protected]>" "Guillaume Melquiond <[email protected]>" ]
synopsis: "(patched for Coq 8.11 compatibility by MSoegtropIMC) A Coq formalization of real analysis compatible with the standard library"
url {
src: "https://github.com/MSoegtropIMC/coquelicot/archive/1ec80657ce992fc5aa660dca86d423671f02e33c.tar.gz"
checksum: "sha512=c164168d3732ff9f0067e95997d6478be497244380a297ac8c092ebefa2d369246f4f8a1e6ae7d5ec6fd32e73f3b9cf96addd067b80b5bc67f3883e0933a3ce2"
}
</pre>
<h2>Lint</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Dry install 🏜️</h2>
<p>Dry install with the current Coq version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam install -y --show-action coq-coquelicot.3.0.3+8.11 coq.8.8.2</code></dd>
<dt>Return code</dt>
<dd>5120</dd>
<dt>Output</dt>
<dd><pre>[NOTE] Package coq is already installed (current version is 8.8.2).
The following dependencies couldn't be met:
- coq-coquelicot -> coq = 8.11.0
Your request can't be satisfied:
- No available version of coq satisfies the constraints
No solution found, exiting
</pre></dd>
</dl>
<p>Dry install without Coq/switch base, to test if the problem was incompatibility with the current Coq/OCaml version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam remove -y coq; opam install -y --show-action --unlock-base coq-coquelicot.3.0.3+8.11</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Install dependencies</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>0 s</dd>
</dl>
<h2>Install 🚀</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>0 s</dd>
</dl>
<h2>Installation size</h2>
<p>No files were installed.</p>
<h2>Uninstall 🧹</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Missing removes</dt>
<dd>
none
</dd>
<dt>Wrong removes</dt>
<dd>
none
</dd>
</dl>
</div>
</div>
</div>
<hr/>
<div class="footer">
<p class="text-center">
Sources are on <a href="https://github.com/coq-bench">GitHub</a> © Guillaume Claret 🐣
</p>
</div>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
<script src="../../../../../bootstrap.min.js"></script>
</body>
</html>
| {
"content_hash": "9a654aea5ef20a339b3d42026908db32",
"timestamp": "",
"source": "github",
"line_count": 166,
"max_line_length": 252,
"avg_line_length": 44.006024096385545,
"alnum_prop": 0.5585215605749486,
"repo_name": "coq-bench/coq-bench.github.io",
"id": "bfdc1bd4a8fac713f54f91bb60ed38a49122e9b4",
"size": "7330",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "clean/Linux-x86_64-4.09.1-2.0.6/released/8.8.2/coquelicot/3.0.3+8.11.html",
"mode": "33188",
"license": "mit",
"language": [],
"symlink_target": ""
} |
<?php
namespace app\modules\test\controllers\home;
class DefaultController extends \app\core\web\HomeController
{
public function actionIndex()
{
return $this->render('index');
}
}
| {
"content_hash": "bf0d12523701bc5f6cb8e278fa76cc0f",
"timestamp": "",
"source": "github",
"line_count": 12,
"max_line_length": 60,
"avg_line_length": 17,
"alnum_prop": 0.6862745098039216,
"repo_name": "cboy868/lion2",
"id": "0a658f5a76bab6552fb95140ec13ef596a41921c",
"size": "204",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "modules/test/controllers/home/DefaultController.php",
"mode": "33188",
"license": "bsd-3-clause",
"language": [
{
"name": "Batchfile",
"bytes": "515"
},
{
"name": "CSS",
"bytes": "583055"
},
{
"name": "HTML",
"bytes": "1199134"
},
{
"name": "JavaScript",
"bytes": "4579440"
},
{
"name": "PHP",
"bytes": "1054431"
}
],
"symlink_target": ""
} |
package com.amazonaws.services.mediaconvert.model.transform;
import java.util.List;
import javax.annotation.Generated;
import com.amazonaws.SdkClientException;
import com.amazonaws.services.mediaconvert.model.*;
import com.amazonaws.protocol.*;
import com.amazonaws.annotation.SdkInternalApi;
/**
* AutomatedAbrSettingsMarshaller
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
@SdkInternalApi
public class AutomatedAbrSettingsMarshaller {
private static final MarshallingInfo<Integer> MAXABRBITRATE_BINDING = MarshallingInfo.builder(MarshallingType.INTEGER)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("maxAbrBitrate").build();
private static final MarshallingInfo<Integer> MAXRENDITIONS_BINDING = MarshallingInfo.builder(MarshallingType.INTEGER)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("maxRenditions").build();
private static final MarshallingInfo<Integer> MINABRBITRATE_BINDING = MarshallingInfo.builder(MarshallingType.INTEGER)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("minAbrBitrate").build();
private static final MarshallingInfo<List> RULES_BINDING = MarshallingInfo.builder(MarshallingType.LIST).marshallLocation(MarshallLocation.PAYLOAD)
.marshallLocationName("rules").build();
private static final AutomatedAbrSettingsMarshaller instance = new AutomatedAbrSettingsMarshaller();
public static AutomatedAbrSettingsMarshaller getInstance() {
return instance;
}
/**
* Marshall the given parameter object.
*/
public void marshall(AutomatedAbrSettings automatedAbrSettings, ProtocolMarshaller protocolMarshaller) {
if (automatedAbrSettings == null) {
throw new SdkClientException("Invalid argument passed to marshall(...)");
}
try {
protocolMarshaller.marshall(automatedAbrSettings.getMaxAbrBitrate(), MAXABRBITRATE_BINDING);
protocolMarshaller.marshall(automatedAbrSettings.getMaxRenditions(), MAXRENDITIONS_BINDING);
protocolMarshaller.marshall(automatedAbrSettings.getMinAbrBitrate(), MINABRBITRATE_BINDING);
protocolMarshaller.marshall(automatedAbrSettings.getRules(), RULES_BINDING);
} catch (Exception e) {
throw new SdkClientException("Unable to marshall request to JSON: " + e.getMessage(), e);
}
}
}
| {
"content_hash": "bcb266613e0dc2f9d750b201bad182f4",
"timestamp": "",
"source": "github",
"line_count": 54,
"max_line_length": 151,
"avg_line_length": 44.94444444444444,
"alnum_prop": 0.7581376184590028,
"repo_name": "aws/aws-sdk-java",
"id": "81c921bd8e985cf6f851959514c8cd0bdc16b96d",
"size": "3007",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "aws-java-sdk-mediaconvert/src/main/java/com/amazonaws/services/mediaconvert/model/transform/AutomatedAbrSettingsMarshaller.java",
"mode": "33188",
"license": "apache-2.0",
"language": [],
"symlink_target": ""
} |
using OpenQA.Selenium;
namespace ExtjsWd.Elements
{
public class BasicComboBox : ComboBox<BasicComboBox>
{
public BasicComboBox(IWebDriver driver, By selector)
: base(driver, selector)
{
}
public BasicComboBox(IWebDriver driver, string cssClass)
: base(driver, cssClass)
{
}
public BasicComboBox(IWebDriver driver, int timeoutInSeconds)
: base(driver, timeoutInSeconds)
{
}
public override BasicComboBox FillIn(string value)
{
Input.SendKeys(value);
return this;
}
}
} | {
"content_hash": "2e5b80e15bba4dba2e20371e3c554faf",
"timestamp": "",
"source": "github",
"line_count": 28,
"max_line_length": 69,
"avg_line_length": 22.892857142857142,
"alnum_prop": 0.5772230889235569,
"repo_name": "pratoservices/extjswebdriver",
"id": "34f4fec47466c7c7ad3ed1595f93cfe055e11b32",
"size": "643",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "ExtjsWd/Elements/BasicComboBox.cs",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "Batchfile",
"bytes": "36"
},
{
"name": "C#",
"bytes": "67849"
},
{
"name": "HTML",
"bytes": "436"
},
{
"name": "JavaScript",
"bytes": "1365"
}
],
"symlink_target": ""
} |
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Net;
using System.IO;
namespace ZencoderDotNet
{
public class Request
{
// update the request object whenever a public field is changed
private string requestBody;
public string body
{
get { return requestBody; }
set
{
requestBody = value;
setupRequest();
}
}
private string requestMethod;
public string method
{
get { return requestMethod; }
set
{
requestMethod = value;
setupRequest();
}
}
private string requestFormat;
public string format
{
get
{
if (requestFormat == null)
{
return Zencoder.format;
}
else
{
return requestFormat;
}
}
set
{
requestFormat = value;
setupRequest();
}
}
private string requestUrl;
public string url
{
get
{
return requestUrl;
}
set
{
requestUrl = value;
setupRequest();
}
}
private HttpWebRequest webRequest;
public Request(string Url, string Method, string Body = null, string Format = null)
{
// Setting private variables directly so setupRequest() isn't called each time
requestUrl = Url;
requestBody = Body;
requestMethod = Method;
requestFormat = Format;
setupRequest();
}
public Response getResponse()
{
HttpWebResponse response = (HttpWebResponse)webRequest.GetResponse();
return new Response(response, format);
}
private void setupRequest()
{
webRequest = (HttpWebRequest)WebRequest.Create(Zencoder.base_url + "/" + requestUrl);
webRequest.Proxy = null;
webRequest.Method = method;
setRequestHeaders();
if (body != null && method != "GET")
{
setRequestBody();
}
}
private void setRequestHeaders()
{
if (format == "xml")
{
webRequest.Accept = "application/xml";
webRequest.ContentType = "application/xml";
}
else
{
webRequest.Accept = "application/json";
webRequest.ContentType = "application/json";
}
webRequest.Headers.Add("Zencoder-Library-Name: " + Zencoder.library_name);
webRequest.Headers.Add("Zencoder-Library-Version: " + Zencoder.library_version);
}
private void setRequestBody()
{
byte[] reqData = Encoding.UTF8.GetBytes(body);
webRequest.ContentLength = reqData.Length;
using (Stream reqStream = webRequest.GetRequestStream())
{
reqStream.Write(reqData, 0, reqData.Length);
}
}
}
}
| {
"content_hash": "8c22e1272be64fd92498c59d393cb870",
"timestamp": "",
"source": "github",
"line_count": 128,
"max_line_length": 97,
"avg_line_length": 26.6953125,
"alnum_prop": 0.4717588527948493,
"repo_name": "zencoder/zencoder-dotnet",
"id": "4506513a50bbc4a1db9ad4c3ee3dbdf9cdee0839",
"size": "3419",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "zencoder-dotnet/Request.cs",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "C#",
"bytes": "18941"
}
],
"symlink_target": ""
} |
//
// SPUserManager.m
// sequel-pro
//
// Created by Mark Townsend on Jan 1, 2009.
// Copyright (c) 2009 Mark Townsend. All rights reserved.
//
// Permission is hereby granted, free of charge, to any person
// obtaining a copy of this software and associated documentation
// files (the "Software"), to deal in the Software without
// restriction, including without limitation the rights to use,
// copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the
// Software is furnished to do so, subject to the following
// conditions:
//
// The above copyright notice and this permission notice shall be
// included in all copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
// EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES
// OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
// NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
// HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY,
// WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
// FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR
// OTHER DEALINGS IN THE SOFTWARE.
//
// More info at <https://github.com/sequelpro/sequelpro>
#import "SPUserManager.h"
#import "SPUserMO.h"
#import "SPPrivilegesMO.h"
#import "ImageAndTextCell.h"
#import "SPGrowlController.h"
#import "SPConnectionController.h"
#import "SPServerSupport.h"
#import "SPAlertSheets.h"
#import "SPSplitView.h"
#import "SPDatabaseDocument.h"
#import <SPMySQL/SPMySQL.h>
#import <QueryKit/QueryKit.h>
static NSString * const SPTableViewNameColumnID = @"NameColumn";
@interface SPUserManager ()
- (void)_initializeTree:(NSArray *)items;
- (void)_initializeUsers;
- (void)_selectParentFromSelection;
- (NSArray *)_fetchUserWithUserName:(NSString *)username;
- (SPUserMO *)_createNewSPUser;
- (BOOL)_grantPrivileges:(NSArray *)thePrivileges onDatabase:(NSString *)aDatabase forUser:(NSString *)aUser host:(NSString *)aHost;
- (BOOL)_revokePrivileges:(NSArray *)thePrivileges onDatabase:(NSString *)aDatabase forUser:(NSString *)aUser host:(NSString *)aHost;
- (BOOL)_checkAndDisplayMySqlError;
- (void)_clearData;
- (void)_initializeChild:(NSManagedObject *)child withItem:(NSDictionary *)item;
- (void)_initializeSchemaPrivsForChild:(SPUserMO *)child fromData:(NSArray *)dataForUser;
- (void)_initializeSchemaPrivs;
- (NSArray *)_fetchPrivsWithUser:(NSString *)username schema:(NSString *)selectedSchema host:(NSString *)host;
- (void)_setSchemaPrivValues:(NSArray *)objects enabled:(BOOL)enabled;
- (void)_initializeAvailablePrivs;
- (BOOL)_renameUserFrom:(NSString *)originalUser host:(NSString *)originalHost to:(NSString *)newUser host:(NSString *)newHost;
- (void)sheetDidEnd:(NSWindow *)sheet returnCode:(int)returnCode contextInfo:(void*)context;
- (void)contextWillSave:(NSNotification *)notice;
@end
@implementation SPUserManager
@synthesize connection;
@synthesize databaseDocument;
@synthesize privsSupportedByServer;
@synthesize managedObjectContext;
@synthesize managedObjectModel;
@synthesize persistentStoreCoordinator;
@synthesize schemas;
@synthesize grantedSchemaPrivs;
@synthesize availablePrivs;
@synthesize treeSortDescriptors;
@synthesize serverSupport;
@synthesize isInitializing = isInitializing;
#pragma mark -
#pragma mark Initialisation
- (id)init
{
if ((self = [super initWithWindowNibName:@"UserManagerView"])) {
// When reading privileges from the database, they are converted automatically to a
// lowercase key used in the user privileges stores, from which a GRANT syntax
// is derived automatically. While most keys can be automatically converted without
// any difficulty, some keys differ slightly in mysql column storage to GRANT syntax;
// this dictionary provides mappings for those values to ensure consistency.
privColumnToGrantMap = [@{
@"Grant_priv": @"Grant_option_priv",
@"Show_db_priv": @"Show_databases_priv",
@"Create_tmp_table_priv": @"Create_temporary_tables_priv",
@"Repl_slave_priv": @"Replication_slave_priv",
@"Repl_client_priv": @"Replication_client_priv",
} retain];
schemas = [[NSMutableArray alloc] init];
availablePrivs = [[NSMutableArray alloc] init];
grantedSchemaPrivs = [[NSMutableArray alloc] init];
isSaving = NO;
}
return self;
}
/**
* UI specific items to set up when the window loads. This is different than awakeFromNib
* as it's only called once.
*/
- (void)windowDidLoad
{
[tabView selectTabViewItemAtIndex:0];
[splitView setMinSize:120.f ofSubviewAtIndex:0];
[splitView setMinSize:620.f ofSubviewAtIndex:1];
NSTableColumn *tableColumn = [outlineView tableColumnWithIdentifier:SPTableViewNameColumnID];
ImageAndTextCell *imageAndTextCell = [[[ImageAndTextCell alloc] init] autorelease];
[imageAndTextCell setEditable:NO];
[tableColumn setDataCell:imageAndTextCell];
// Set schema table double-click actions
[grantedTableView setDoubleAction:@selector(doubleClickSchemaPriv:)];
[availableTableView setDoubleAction:@selector(doubleClickSchemaPriv:)];
[self _initializeSchemaPrivs];
[self _initializeUsers];
[self _initializeAvailablePrivs];
treeSortDescriptor = [[NSSortDescriptor alloc] initWithKey:@"displayName" ascending:YES];
[self setTreeSortDescriptors:@[treeSortDescriptor]];
[super windowDidLoad];
}
/**
* This method reads in the users from the mysql.user table of the current
* connection. Then uses this information to initialize the NSOutlineView.
*/
- (void)_initializeUsers
{
isInitializing = YES; // Don't want to do some of the notifications if initializing
NSMutableString *privKey;
NSArray *privRow;
NSAutoreleasePool *pool = [[NSAutoreleasePool alloc] init];
NSMutableArray *usersResultArray = [NSMutableArray array];
// Select users from the mysql.user table
SPMySQLResult *result = [connection queryString:@"SELECT * FROM mysql.user ORDER BY user"];
[result setReturnDataAsStrings:YES];
//TODO: improve user feedback
NSAssert(([[result fieldNames] firstObjectCommonWithArray:@[@"Password",@"authentication_string"]] != nil), @"Resultset from mysql.user contains neither 'Password' nor 'authentication_string' column!?");
requiresPost576PasswordHandling = ![[result fieldNames] containsObject:@"Password"];
[usersResultArray addObjectsFromArray:[result getAllRows]];
[self _initializeTree:usersResultArray];
// Set up the array of privs supported by this server.
[[self privsSupportedByServer] removeAllObjects];
result = nil;
// Attempt to obtain user privileges if supported
if ([serverSupport supportsShowPrivileges]) {
result = [connection queryString:@"SHOW PRIVILEGES"];
[result setReturnDataAsStrings:YES];
}
if (result && [result numberOfRows]) {
while ((privRow = [result getRowAsArray]))
{
privKey = [NSMutableString stringWithString:[[privRow objectAtIndex:0] lowercaseString]];
// Skip the special "Usage" key
if ([privKey isEqualToString:@"usage"]) continue;
[privKey replaceOccurrencesOfString:@" " withString:@"_" options:NSLiteralSearch range:NSMakeRange(0, [privKey length])];
[privKey appendString:@"_priv"];
[[self privsSupportedByServer] setValue:@YES forKey:privKey];
}
}
// If that fails, base privilege support on the mysql.users columns
else {
result = [connection queryString:@"SHOW COLUMNS FROM mysql.user"];
[result setReturnDataAsStrings:YES];
while ((privRow = [result getRowAsArray]))
{
privKey = [NSMutableString stringWithString:[privRow objectAtIndex:0]];
if (![privKey hasSuffix:@"_priv"]) continue;
if ([privColumnToGrantMap objectForKey:privKey]) privKey = [privColumnToGrantMap objectForKey:privKey];
[[self privsSupportedByServer] setValue:@YES forKey:[privKey lowercaseString]];
}
}
[pool release];
isInitializing = NO;
}
/**
* Initialize the outline view tree. The NSOutlineView gets it's data from a NSTreeController which gets
* it's data from the SPUser Entity objects in the current managedObjectContext.
*/
- (void)_initializeTree:(NSArray *)items
{
// Retrieve all the user data in order to be able to initialise the schema privs for each child,
// copying into a dictionary keyed by user, each with all the host rows.
NSMutableDictionary *schemaPrivilegeData = [NSMutableDictionary dictionary];
SPMySQLResult *queryResults = [connection queryString:@"SELECT * FROM mysql.db"];
[queryResults setReturnDataAsStrings:YES];
for (NSDictionary *privRow in queryResults)
{
if (![schemaPrivilegeData objectForKey:[privRow objectForKey:@"User"]]) {
[schemaPrivilegeData setObject:[NSMutableArray array] forKey:[privRow objectForKey:@"User"]];
}
[[schemaPrivilegeData objectForKey:[privRow objectForKey:@"User"]] addObject:privRow];
// If "all database" values were found, add them to the schemas list if not already present
NSString *schemaName = [privRow objectForKey:@"Db"];
if ([schemaName isEqualToString:@""] || [schemaName isEqualToString:@"%"]) {
if (![schemas containsObject:schemaName]) {
[schemas addObject:schemaName];
[schemasTableView noteNumberOfRowsChanged];
}
}
}
// Go through each item that contains a dictionary of key-value pairs
// for each user currently in the database.
for (NSUInteger i = 0; i < [items count]; i++)
{
NSDictionary *item = [items objectAtIndex:i];
NSString *username = [item objectForKey:@"User"];
NSArray *parentResults = [[self _fetchUserWithUserName:username] retain];
SPUserMO *parent;
SPUserMO *child;
// Check to make sure if we already have added the parent
if (parentResults != nil && [parentResults count] > 0) {
// Add Children
parent = [parentResults objectAtIndex:0];
child = [self _createNewSPUser];
}
else {
// Add Parent
parent = [self _createNewSPUser];
child = [self _createNewSPUser];
// We only care about setting the user and password keys on the parent, together with their
// original values for comparison purposes
[parent setPrimitiveValue:username forKey:@"user"];
[parent setPrimitiveValue:username forKey:@"originaluser"];
if (requiresPost576PasswordHandling) {
[parent setPrimitiveValue:[item objectForKey:@"plugin"] forKey:@"plugin"];
NSString *passwordHash = [item objectForKey:@"authentication_string"];
if (![passwordHash isNSNull]) {
[parent setPrimitiveValue:passwordHash forKey:@"authentication_string"];
// for the UI dialog
if ([passwordHash length]) {
[parent setPrimitiveValue:@"sequelpro_dummy_password" forKey:@"password"];
}
}
}
else {
[parent setPrimitiveValue:[item objectForKey:@"Password"] forKey:@"password"];
[parent setPrimitiveValue:[item objectForKey:@"Password"] forKey:@"originalpassword"];
}
}
// Setup the NSManagedObject with values from the dictionary
[self _initializeChild:child withItem:item];
NSMutableSet *children = [parent mutableSetValueForKey:@"children"];
[children addObject:child];
[self _initializeSchemaPrivsForChild:child fromData:[schemaPrivilegeData objectForKey:username]];
// Save the initialized objects so that any new changes will be tracked.
NSError *error = nil;
[[self managedObjectContext] save:&error];
if (error != nil) {
[NSApp presentError:error];
}
[parentResults release];
}
// Reload data of the outline view with the changes.
[outlineView reloadData];
[treeController rearrangeObjects];
}
/**
* Initialize the available user privileges.
*/
- (void)_initializeAvailablePrivs
{
// Initialize available privileges
NSManagedObjectContext *moc = [self managedObjectContext];
NSEntityDescription *privEntityDescription = [NSEntityDescription entityForName:@"Privileges" inManagedObjectContext:moc];
NSArray *props = [privEntityDescription attributeKeys];
[availablePrivs removeAllObjects];
for (NSString *prop in props)
{
if ([prop hasSuffix:@"_priv"] && [[[self privsSupportedByServer] objectForKey:prop] boolValue]) {
NSString *displayName = [[prop stringByReplacingOccurrencesOfString:@"_priv" withString:@""] replaceUnderscoreWithSpace];
[availablePrivs addObject:[NSDictionary dictionaryWithObjectsAndKeys:displayName, @"displayName", prop, @"name", nil]];
}
}
[availableController rearrangeObjects];
}
/**
* Initialize the available schema privileges.
*/
- (void)_initializeSchemaPrivs
{
// Initialize Databases
[schemas removeAllObjects];
[schemas addObjectsFromArray:[databaseDocument allDatabaseNames]];
[schemasTableView reloadData];
}
/**
* Set NSManagedObject with values from the passed in dictionary.
*/
- (void)_initializeChild:(NSManagedObject *)child withItem:(NSDictionary *)item
{
for (NSString *key in item)
{
// In order to keep the priviledges a little more dynamic, just
// go through the keys that have the _priv suffix. If a priviledge is
// currently not supported in the model, then an exception is thrown.
// We catch that exception and print to the console for future enhancement.
NS_DURING
if ([key hasSuffix:@"_priv"])
{
BOOL value = [[item objectForKey:key] boolValue];
// Special case keys
if ([privColumnToGrantMap objectForKey:key])
{
key = [privColumnToGrantMap objectForKey:key];
}
[child setValue:[NSNumber numberWithBool:value] forKey:key];
}
else if ([key hasPrefix:@"max"]) // Resource Management restrictions
{
NSNumber *value = [NSNumber numberWithInteger:[[item objectForKey:key] integerValue]];
[child setValue:value forKey:key];
}
else if (![key isInArray:@[@"User",@"Password",@"plugin",@"authentication_string"]])
{
NSString *value = [item objectForKey:key];
[child setValue:value forKey:key];
}
NS_HANDLER
NS_ENDHANDLER
}
}
/**
* Initialize the schema privileges for the supplied child object.
*
* Assumes that the child has already been initialized with values from the
* global user table.
*/
- (void)_initializeSchemaPrivsForChild:(SPUserMO *)child fromData:(NSArray *)dataForUser
{
NSMutableSet *privs = [child mutableSetValueForKey:@"schema_privileges"];
// Set an originalhost key on the child to allow the tracking of edits
[child setPrimitiveValue:[child valueForKey:@"host"] forKey:@"originalhost"];
for (NSDictionary *rowDict in dataForUser)
{
// Verify that the host matches, or skip this entry
if (![[rowDict objectForKey:@"Host"] isEqualToString:[child valueForKey:@"host"]]) {
continue;
}
SPPrivilegesMO *dbPriv = [NSEntityDescription insertNewObjectForEntityForName:@"Privileges" inManagedObjectContext:[self managedObjectContext]];
for (NSString *key in rowDict)
{
if ([key hasSuffix:@"_priv"]) {
BOOL boolValue = [[rowDict objectForKey:key] boolValue];
// Special case keys
if ([privColumnToGrantMap objectForKey:key]) {
key = [privColumnToGrantMap objectForKey:key];
}
[dbPriv setValue:[NSNumber numberWithBool:boolValue] forKey:key];
}
else if ([key isEqualToString:@"Db"]) {
NSString *db = [[rowDict objectForKey:key] stringByReplacingOccurrencesOfString:@"\\_" withString:@"_"];
[dbPriv setValue:db forKey:key];
}
else if (![key isEqualToString:@"Host"] && ![key isEqualToString:@"User"]) {
[dbPriv setValue:[rowDict objectForKey:key] forKey:key];
}
}
[privs addObject:dbPriv];
}
}
/**
* Creates, retains, and returns the managed object model for the application
* by merging all of the models found in the application bundle.
*/
- (NSManagedObjectModel *)managedObjectModel
{
if (!managedObjectModel) {
managedObjectModel = [[NSManagedObjectModel mergedModelFromBundles:nil] retain];
}
return managedObjectModel;
}
/**
* Returns the persistent store coordinator for the application. This
* implementation will create and return a coordinator, having added the
* store for the application to it. (The folder for the store is created,
* if necessary.)
*/
- (NSPersistentStoreCoordinator *)persistentStoreCoordinator
{
if (persistentStoreCoordinator != nil) return persistentStoreCoordinator;
NSError *error = nil;
persistentStoreCoordinator = [[NSPersistentStoreCoordinator alloc] initWithManagedObjectModel: [self managedObjectModel]];
if (![persistentStoreCoordinator addPersistentStoreWithType:NSInMemoryStoreType configuration:nil URL:nil options:nil error:&error] && error) {
[NSApp presentError:error];
}
return persistentStoreCoordinator;
}
/**
* Returns the managed object context for the application (which is already
* bound to the persistent store coordinator for the application.)
*/
- (NSManagedObjectContext *)managedObjectContext
{
if (managedObjectContext != nil) return managedObjectContext;
NSPersistentStoreCoordinator *coordinator = [self persistentStoreCoordinator];
if (coordinator != nil) {
managedObjectContext = [[NSManagedObjectContext alloc] init];
[managedObjectContext setPersistentStoreCoordinator:coordinator];
}
[[NSNotificationCenter defaultCenter] addObserver:self
selector:@selector(contextWillSave:)
name:NSManagedObjectContextWillSaveNotification
object:managedObjectContext];
return managedObjectContext;
}
- (void)beginSheetModalForWindow:(NSWindow *)docWindow completionHandler:(void (^)())callback
{
//copy block from stack to heap, otherwise it wouldn't live long enough to be invoked later.
void *heapCallback = callback? Block_copy(callback) : NULL;
[NSApp beginSheet:[self window]
modalForWindow:docWindow
modalDelegate:self
didEndSelector:@selector(sheetDidEnd:returnCode:contextInfo:)
contextInfo:heapCallback];
}
- (void)sheetDidEnd:(NSWindow *)sheet returnCode:(int)returnCode contextInfo:(void*)context
{
//[NSApp endSheet...] does not close the window
[[self window] orderOut:self];
//notify delegate
if(context) {
void (^callback)() = context;
//directly invoking callback would risk that we are dealloc'd while still in this run loop iteration.
dispatch_async(dispatch_get_main_queue(), callback);
Block_release(callback);
}
}
#pragma mark -
#pragma mark General IBAction methods
/**
* Closes the user manager and reverts any changes made.
*/
- (IBAction)doCancel:(id)sender
{
// Discard any pending changes
[treeController discardEditing];
// Change the first responder to end editing in any field
[[self window] makeFirstResponder:self];
[[self managedObjectContext] rollback];
// Close sheet
[NSApp endSheet:[self window] returnCode:0];
}
/**
* Closes the user manager and applies any changes made.
*/
- (IBAction)doApply:(id)sender
{
// If editing can't be committed, cancel the apply
if (![treeController commitEditing]) {
return;
}
errorsString = [[NSMutableString alloc] init];
// Change the first responder to end editing in any field
[[self window] makeFirstResponder:self];
isSaving = YES;
NSError *error = nil;
[[self managedObjectContext] save:&error];
isSaving = NO;
if (error) [errorsString appendString:[error localizedDescription]];
[connection queryString:@"FLUSH PRIVILEGES"];
// Display any errors
if ([errorsString length]) {
[errorsTextView setString:errorsString];
[NSApp beginSheet:errorsSheet
modalForWindow:[NSApp keyWindow]
modalDelegate:nil
didEndSelector:NULL
contextInfo:nil];
SPClear(errorsString);
return;
}
SPClear(errorsString);
// Otherwise, close the sheet
[NSApp endSheet:[self window] returnCode:0];
}
/**
* Enables all privileges.
*/
- (IBAction)checkAllPrivileges:(id)sender
{
id selectedUser = [[treeController selectedObjects] objectAtIndex:0];
// Iterate through the supported privs, setting the value of each to YES
for (NSString *key in [self privsSupportedByServer])
{
if (![key hasSuffix:@"_priv"]) continue;
// Perform the change in a try/catch check to avoid exceptions for unhandled privs
NS_DURING
[selectedUser setValue:@YES forKey:key];
NS_HANDLER
NS_ENDHANDLER
}
}
/**
* Disables all privileges.
*/
- (IBAction)uncheckAllPrivileges:(id)sender
{
id selectedUser = [[treeController selectedObjects] objectAtIndex:0];
// Iterate through the supported privs, setting the value of each to NO
for (NSString *key in [self privsSupportedByServer])
{
if (![key hasSuffix:@"_priv"]) continue;
// Perform the change in a try/catch check to avoid exceptions for unhandled privs
NS_DURING
[selectedUser setValue:@NO forKey:key];
NS_HANDLER
NS_ENDHANDLER
}
}
/**
* Adds a new user to the current database.
*/
- (IBAction)addUser:(id)sender
{
// Adds a new SPUser objects to the managedObjectContext and sets default values
if ([[treeController selectedObjects] count] > 0) {
if ([[[treeController selectedObjects] objectAtIndex:0] parent] != nil) {
[self _selectParentFromSelection];
}
}
SPUserMO *newItem = [self _createNewSPUser];
SPUserMO *newChild = [self _createNewSPUser];
[newChild setValue:@"localhost" forKey:@"host"];
[newItem addChildrenObject:newChild];
[treeController addObject:newItem];
[outlineView expandItem:[outlineView itemAtRow:[outlineView selectedRow]]];
[[self window] makeFirstResponder:userNameTextField];
}
/**
* Removes the currently selected user from the current database.
*/
- (IBAction)removeUser:(id)sender
{
NSString *username = [[[treeController selectedObjects] objectAtIndex:0] valueForKey:@"originaluser"];
NSArray *children = [[[treeController selectedObjects] objectAtIndex:0] valueForKey:@"children"];
// On all the children - host entries - set the username to be deleted,
// for later query contruction.
for (NSManagedObject *child in children)
{
[child setPrimitiveValue:username forKey:@"user"];
}
// Unset the host on the user, so that only the host entries are dropped
[[[treeController selectedObjects] objectAtIndex:0] setPrimitiveValue:nil forKey:@"host"];
[treeController remove:sender];
}
/**
* Adds a new host to the currently selected user.
*/
- (IBAction)addHost:(id)sender
{
if ([[treeController selectedObjects] count] > 0)
{
if ([[[treeController selectedObjects] objectAtIndex:0] parent] != nil)
{
[self _selectParentFromSelection];
}
}
[treeController addChild:sender];
// The newly added item will be selected as it is added, but only after the next iteration of the
// run loop - edit it after a tiny delay.
[self performSelector:@selector(editNewHost) withObject:nil afterDelay:0.1];
}
/**
* Perform a deferred edit of the currently selected row.
*/
- (void)editNewHost
{
[outlineView editColumn:0 row:[outlineView selectedRow] withEvent:nil select:YES];
}
/**
* Removes the currently selected host from it's parent user.
*/
- (IBAction)removeHost:(id)sender
{
// Set the username on the child so that it's accessabile when building
// the drop sql command
SPUserMO *child = [[treeController selectedObjects] objectAtIndex:0];
SPUserMO *parent = [child parent];
[child setPrimitiveValue:[[child valueForKey:@"parent"] valueForKey:@"user"] forKey:@"user"];
[treeController remove:sender];
if ([[parent valueForKey:@"children"] count] == 0)
{
SPOnewayAlertSheet(
NSLocalizedString(@"Unable to remove host", @"error removing host message"),
[self window],
NSLocalizedString(@"This user doesn't seem to have any associated hosts and will be removed unless a host is added.", @"error removing host informative message")
);
}
}
/**
* Adds a new schema privilege.
*/
- (IBAction)addSchemaPriv:(id)sender
{
NSArray *selectedObjects = [availableController selectedObjects];
[grantedController addObjects:selectedObjects];
[grantedTableView noteNumberOfRowsChanged];
[availableController removeObjects:selectedObjects];
[availableTableView noteNumberOfRowsChanged];
[schemasTableView setNeedsDisplay:YES];
[self _setSchemaPrivValues:selectedObjects enabled:YES];
}
/**
* Removes a schema privilege.
*/
- (IBAction)removeSchemaPriv:(id)sender
{
NSArray *selectedObjects = [grantedController selectedObjects];
[availableController addObjects:selectedObjects];
[availableTableView noteNumberOfRowsChanged];
[grantedController removeObjects:selectedObjects];
[grantedTableView noteNumberOfRowsChanged];
[schemasTableView setNeedsDisplay:YES];
[self _setSchemaPrivValues:selectedObjects enabled:NO];
}
/**
* Move double-clicked rows across to the other table, using the
* appropriate methods.
*/
- (IBAction)doubleClickSchemaPriv:(id)sender
{
// Ignore double-clicked header cells
if ([sender clickedRow] == -1) return;
if (sender == availableTableView) {
[self addSchemaPriv:sender];
}
else {
[self removeSchemaPriv:sender];
}
}
/**
* Refreshes the current list of users.
*/
- (IBAction)refresh:(id)sender
{
if ([[self managedObjectContext] hasChanges]) {
NSAlert *alert = [NSAlert alertWithMessageText:NSLocalizedString(@"Unsaved changes", @"unsaved changes message")
defaultButton:NSLocalizedString(@"Continue", @"continue button")
alternateButton:NSLocalizedString(@"Cancel", @"cancel button")
otherButton:nil
informativeTextWithFormat:NSLocalizedString(@"Changes have been made, which will be lost if this window is closed. Are you sure you want to continue", @"unsaved changes informative message")];
[alert setAlertStyle:NSWarningAlertStyle];
// Cancel
if ([alert runModal] == NSAlertAlternateReturn) return;
}
[[self managedObjectContext] reset];
[grantedSchemaPrivs removeAllObjects];
[grantedTableView reloadData];
[self _initializeAvailablePrivs];
[outlineView reloadData];
[treeController rearrangeObjects];
// Get all the stores on the current MOC and remove them.
NSArray *stores = [[[self managedObjectContext] persistentStoreCoordinator] persistentStores];
for (NSPersistentStore* store in stores)
{
[[[self managedObjectContext] persistentStoreCoordinator] removePersistentStore:store error:nil];
}
// Add a new store
[[[self managedObjectContext] persistentStoreCoordinator] addPersistentStoreWithType:NSInMemoryStoreType configuration:nil URL:nil options:nil error:nil];
// Reinitialize the tree with values from the database.
[self _initializeUsers];
// After the reset, ensure all original password and user values are up-to-date.
NSEntityDescription *entityDescription = [NSEntityDescription entityForName:@"SPUser" inManagedObjectContext:[self managedObjectContext]];
NSFetchRequest *request = [[[NSFetchRequest alloc] init] autorelease];
[request setEntity:entityDescription];
NSArray *userArray = [[self managedObjectContext] executeFetchRequest:request error:nil];
for (SPUserMO *user in userArray)
{
if (![user parent]) {
[user setPrimitiveValue:[user valueForKey:@"user"] forKey:@"originaluser"];
if(!requiresPost576PasswordHandling) [user setPrimitiveValue:[user valueForKey:@"password"] forKey:@"originalpassword"];
}
}
}
- (void)_setSchemaPrivValues:(NSArray *)objects enabled:(BOOL)enabled
{
// The passed in objects should be an array of NSDictionaries with a key
// of "name".
NSManagedObject *selectedHost = [[treeController selectedObjects] objectAtIndex:0];
NSString *selectedDb = [schemas objectAtIndex:[schemasTableView selectedRow]];
NSArray *selectedPrivs = [self _fetchPrivsWithUser:[selectedHost valueForKeyPath:@"parent.user"]
schema:[selectedDb stringByReplacingOccurrencesOfString:@"_" withString:@"\\_"]
host:[selectedHost valueForKey:@"host"]];
BOOL isNew = NO;
NSManagedObject *priv = nil;
if ([selectedPrivs count] > 0){
priv = [selectedPrivs objectAtIndex:0];
}
else {
priv = [NSEntityDescription insertNewObjectForEntityForName:@"Privileges" inManagedObjectContext:[self managedObjectContext]];
[priv setValue:selectedDb forKey:@"db"];
isNew = YES;
}
// Now setup all the items that are selected to their enabled value
for (NSDictionary *obj in objects)
{
[priv setValue:[NSNumber numberWithBool:enabled] forKey:[obj valueForKey:@"name"]];
}
if (isNew) {
// Set up relationship
NSMutableSet *privs = [selectedHost mutableSetValueForKey:@"schema_privileges"];
[privs addObject:priv];
}
}
- (void)_clearData
{
[managedObjectContext reset];
SPClear(managedObjectContext);
}
/**
* Menu item validation.
*/
- (BOOL)validateMenuItem:(NSMenuItem *)menuItem
{
// Only allow removing hosts of a host node is selected.
if ([menuItem action] == @selector(removeHost:)) {
return (([[treeController selectedObjects] count] > 0) &&
[[[treeController selectedObjects] objectAtIndex:0] parent] != nil);
}
else if ([menuItem action] == @selector(addHost:)) {
return ([[treeController selectedObjects] count] > 0);
}
return YES;
}
- (void)_selectParentFromSelection
{
if ([[treeController selectedObjects] count] > 0)
{
NSTreeNode *firstSelectedNode = [[treeController selectedNodes] objectAtIndex:0];
NSTreeNode *parentNode = [firstSelectedNode parentNode];
if (parentNode) {
NSIndexPath *parentIndex = [parentNode indexPath];
[treeController setSelectionIndexPath:parentIndex];
}
else {
NSArray *selectedIndexPaths = [treeController selectionIndexPaths];
[treeController removeSelectionIndexPaths:selectedIndexPaths];
}
}
}
- (void)_selectFirstChildOfParentNode
{
if ([[treeController selectedObjects] count] > 0)
{
[outlineView expandItem:[outlineView itemAtRow:[outlineView selectedRow]]];
id selectedObject = [[treeController selectedObjects] objectAtIndex:0];
NSTreeNode *firstSelectedNode = [[treeController selectedNodes] objectAtIndex:0];
id parent = [selectedObject parent];
// If this is already a parent, then parentNode should be null.
// If a child is already selected, then we want to not change the selection
if (!parent) {
NSIndexPath *childIndex = [[[firstSelectedNode childNodes] objectAtIndex:0] indexPath];
[treeController setSelectionIndexPath:childIndex];
}
}
}
/**
* Closes the supplied sheet, before closing the master window.
*/
- (IBAction)closeErrorsSheet:(id)sender
{
[NSApp endSheet:[sender window] returnCode:[sender tag]];
[[sender window] orderOut:self];
}
#pragma mark -
#pragma mark Notifications
/**
* This notification is called when the managedObjectContext save happens.
*
* This will link this class to any newly created objects, so when they do their
* -validateFor(Insert|Update|Delete): call later, they can forward it to this class.
*/
- (void)contextWillSave:(NSNotification *)notice
{
//new objects don't yet know about us (this will also be called the first time an object is loaded from the db)
for (NSManagedObject *o in [managedObjectContext insertedObjects]) {
if([o isKindOfClass:[SPUserMO class]] || [o isKindOfClass:[SPPrivilegesMO class]]) {
[o setValue:self forKey:@"userManager"];
}
}
}
- (void)contextDidChange:(NSNotification *)notification
{
if (!isInitializing) [outlineView reloadData];
}
#pragma mark -
#pragma mark Core data notifications
- (BOOL)updateUser:(SPUserMO *)user
{
if (![user parent]) {
NSArray *hosts = [user valueForKey:@"children"];
// If the user has been changed, update the username on all hosts.
// Don't check for errors, as some hosts may be new.
if (![[user valueForKey:@"user"] isEqualToString:[user valueForKey:@"originaluser"]]) {
for (SPUserMO *child in hosts)
{
[self _renameUserFrom:[user valueForKey:@"originaluser"]
host:[child valueForKey:@"originalhost"] ? [child valueForKey:@"originalhost"] : [child host]
to:[user valueForKey:@"user"]
host:[child host]];
}
}
// If the password has been changed, use the same password on all hosts
if(requiresPost576PasswordHandling) {
// the UI password field is bound to the password field, so this is still where the new plaintext value comes from
NSString *newPass = [[user changedValues] objectForKey:@"password"];
if(newPass) {
// 5.7.6+ can update all users at once
NSMutableString *alterStmt = [NSMutableString stringWithString:@"ALTER USER "];
BOOL first = YES;
for (SPUserMO *child in hosts)
{
if(!first) [alterStmt appendString:@", "];
[alterStmt appendFormat:@"%@@%@ IDENTIFIED WITH %@ BY %@", //note: "BY" -> plaintext, "AS" -> hash
[[user valueForKey:@"user"] tickQuotedString],
[[child host] tickQuotedString],
[[user valueForKey:@"plugin"] tickQuotedString],
(![newPass isNSNull] && [newPass length]) ? [newPass tickQuotedString] : @"''"];
first = NO;
}
[connection queryString:alterStmt];
if(![self _checkAndDisplayMySqlError]) return NO;
}
}
else {
if (![[user valueForKey:@"password"] isEqualToString:[user valueForKey:@"originalpassword"]]) {
for (SPUserMO *child in hosts)
{
NSString *changePasswordStatement = [NSString stringWithFormat:
@"SET PASSWORD FOR %@@%@ = PASSWORD(%@)",
[[user valueForKey:@"user"] tickQuotedString],
[[child host] tickQuotedString],
([user valueForKey:@"password"]) ? [[user valueForKey:@"password"] tickQuotedString] : @"''"];
[connection queryString:changePasswordStatement];
if(![self _checkAndDisplayMySqlError]) return NO;
}
}
}
}
else {
// If the hostname has changed, remane the detail before editing details
if (![[user valueForKey:@"host"] isEqualToString:[user valueForKey:@"originalhost"]]) {
[self _renameUserFrom:[[user parent] valueForKey:@"originaluser"]
host:[user valueForKey:@"originalhost"]
to:[[user parent] valueForKey:@"user"]
host:[user valueForKey:@"host"]];
}
if ([serverSupport supportsUserMaxVars]) {
if(![self updateResourcesForUser:user]) return NO;
}
if(![self grantPrivilegesToUser:user]) return NO;
}
return YES;
}
- (BOOL)deleteUser:(SPUserMO *)user
{
// users without hosts are for display only
if(isInitializing || ![user valueForKey:@"host"]) return YES;
NSString *droppedUser = [NSString stringWithFormat:@"%@@%@", [[user valueForKey:@"user"] tickQuotedString], [[user valueForKey:@"host"] tickQuotedString]];
// Before MySQL 5.0.2 DROP USER just removed users with no privileges, so revoke
// all their privileges first. Also, REVOKE ALL PRIVILEGES was added in MySQL 4.1.2, so use the
// old multiple query approach (damn, I wish there were only one MySQL version!).
if (![serverSupport supportsFullDropUser]) {
[connection queryString:[NSString stringWithFormat:@"REVOKE ALL PRIVILEGES ON *.* FROM %@", droppedUser]];
if(![self _checkAndDisplayMySqlError]) return NO;
[connection queryString:[NSString stringWithFormat:@"REVOKE GRANT OPTION ON *.* FROM %@", droppedUser]];
if(![self _checkAndDisplayMySqlError]) return NO;
}
// DROP USER was added in MySQL 4.1.1
if ([serverSupport supportsDropUser]) {
[connection queryString:[NSString stringWithFormat:@"DROP USER %@", droppedUser]];
}
// Otherwise manually remove the user rows from the mysql.user table
else {
[connection queryString:[NSString stringWithFormat:@"DELETE FROM mysql.user WHERE User = %@ and Host = %@", [[user valueForKey:@"user"] tickQuotedString], [[user valueForKey:@"host"] tickQuotedString]]];
}
return [self _checkAndDisplayMySqlError];
}
- (BOOL)insertUser:(SPUserMO *)user
{
//this is also called during the initialize phase. we don't want to write to the db there.
if(isInitializing) return YES;
NSString *createStatement = nil;
// Note that if the database does not support the use of the CREATE USER statment, then
// we must resort to using GRANT. Doing so means we must specify the privileges and the database
// for which these apply, so make them as restrictive as possible, but then revoke them to get the
// same affect as CREATE USER. That is, a new user with no privleges.
NSString *host = [[user valueForKey:@"host"] tickQuotedString];
if ([user parent] && [[user parent] valueForKey:@"user"] && ([[user parent] valueForKey:@"password"] || [[user parent] valueForKey:@"authentication_string"])) {
NSString *username = [[[user parent] valueForKey:@"user"] tickQuotedString];
NSString *idString;
if(requiresPost576PasswordHandling) {
// there are three situations to cover here:
// 1) host added, parent user unchanged
// 2) host added, parent user password changed
// 3) host added, parent user is new
if([[user parent] valueForKey:@"originaluser"]) {
// 1 & 2: If the parent user already exists we always use the old password hash. if the parent password changes at the same time, updateUser: will take care of it afterwards
NSString *plugin = [[[user parent] valueForKey:@"plugin"] tickQuotedString];
NSString *hash = [[[user parent] valueForKey:@"authentication_string"] tickQuotedString];
idString = [NSString stringWithFormat:@"IDENTIFIED WITH %@ AS %@",plugin,hash];
}
else {
// 3: If the user is new, we take the plaintext password value from the UI
NSString *password = [[[user parent] valueForKey:@"password"] tickQuotedString];
idString = [NSString stringWithFormat:@"IDENTIFIED BY %@",password];
}
}
else {
BOOL passwordIsHash;
NSString *password;
// there are three situations to cover here:
// 1) host added, parent user unchanged
// 2) host added, parent user password changed
// 3) host added, parent user is new
if([[user parent] valueForKey:@"originaluser"]) {
// 1 & 2: If the parent user already exists we always use the old password hash.
// This works because -updateUser: will be called after -insertUser: and update the password for this host, anyway.
passwordIsHash = YES;
password = [[[user parent] valueForKey:@"originalpassword"] tickQuotedString];
}
else {
// 3: If the user is new, we take the plaintext password value from the UI
passwordIsHash = NO;
password = [[[user parent] valueForKey:@"password"] tickQuotedString];
}
idString = [NSString stringWithFormat:@"IDENTIFIED BY %@%@",(passwordIsHash? @"PASSWORD " : @""), password];
}
createStatement = ([serverSupport supportsCreateUser]) ?
[NSString stringWithFormat:@"CREATE USER %@@%@ %@", username, host, idString] :
[NSString stringWithFormat:@"GRANT SELECT ON mysql.* TO %@@%@ %@", username, host, idString];
}
else if ([user parent] && [[user parent] valueForKey:@"user"]) {
NSString *username = [[[user parent] valueForKey:@"user"] tickQuotedString];
createStatement = ([serverSupport supportsCreateUser]) ?
[NSString stringWithFormat:@"CREATE USER %@@%@", username, host] :
[NSString stringWithFormat:@"GRANT SELECT ON mysql.* TO %@@%@", username, host];
}
if (createStatement) {
// Create user in database
[connection queryString:createStatement];
if ([self _checkAndDisplayMySqlError]) {
if ([serverSupport supportsUserMaxVars]) {
if(![self updateResourcesForUser:user]) return NO;
}
// If we created the user with the GRANT statment (MySQL < 5), then revoke the
// privileges we gave the new user.
if(![serverSupport supportsCreateUser]) {
[connection queryString:[NSString stringWithFormat:@"REVOKE SELECT ON mysql.* FROM %@@%@", [[[user parent] valueForKey:@"user"] tickQuotedString], host]];
if (![self _checkAndDisplayMySqlError]) return NO;
}
return [self grantPrivilegesToUser:user skippingRevoke:YES];
}
}
return NO;
}
- (BOOL)grantDbPrivilegesWithPrivilege:(SPPrivilegesMO *)schemaPriv
{
return [self grantDbPrivilegesWithPrivilege:schemaPriv skippingRevoke:NO];
}
/**
* Grant or revoke DB privileges for the supplied user.
*/
- (BOOL)grantDbPrivilegesWithPrivilege:(SPPrivilegesMO *)schemaPriv skippingRevoke:(BOOL)skipRevoke
{
NSMutableArray *grantPrivileges = [NSMutableArray array];
NSMutableArray *revokePrivileges = [NSMutableArray array];
NSString *dbName = [schemaPriv valueForKey:@"db"];
dbName = [dbName stringByReplacingOccurrencesOfString:@"_" withString:@"\\_"];
NSArray *changedKeys = [[schemaPriv changedValues] allKeys];
for (NSString *key in [self privsSupportedByServer])
{
if (![key hasSuffix:@"_priv"]) continue;
//ignore anything that we didn't change
if (![changedKeys containsObject:key]) continue;
NSString *privilege = [key stringByReplacingOccurrencesOfString:@"_priv" withString:@""];
NS_DURING
if ([[schemaPriv valueForKey:key] boolValue] == YES) {
[grantPrivileges addObject:[privilege replaceUnderscoreWithSpace]];
}
else {
[revokePrivileges addObject:[privilege replaceUnderscoreWithSpace]];
}
NS_HANDLER
NS_ENDHANDLER
}
// Grant privileges
if(![self _grantPrivileges:grantPrivileges
onDatabase:dbName
forUser:[schemaPriv valueForKeyPath:@"user.parent.user"]
host:[schemaPriv valueForKeyPath:@"user.host"]]) return NO;
if(!skipRevoke) {
// Revoke privileges
if(![self _revokePrivileges:revokePrivileges
onDatabase:dbName
forUser:[schemaPriv valueForKeyPath:@"user.parent.user"]
host:[schemaPriv valueForKeyPath:@"user.host"]]) return NO;
}
return YES;
}
/**
* Update resource limites for given user
*/
- (BOOL)updateResourcesForUser:(SPUserMO *)user
{
if ([user valueForKey:@"parent"] != nil) {
NSString *updateResourcesStatement = [NSString stringWithFormat:
@"UPDATE mysql.user SET max_questions = %@, max_updates = %@, max_connections = %@ WHERE User = %@ AND Host = %@",
[user valueForKey:@"max_questions"],
[user valueForKey:@"max_updates"],
[user valueForKey:@"max_connections"],
[[[user valueForKey:@"parent"] valueForKey:@"user"] tickQuotedString],
[[user valueForKey:@"host"] tickQuotedString]];
[connection queryString:updateResourcesStatement];
return [self _checkAndDisplayMySqlError];
}
return YES;
}
- (BOOL)grantPrivilegesToUser:(SPUserMO *)user
{
return [self grantPrivilegesToUser:user skippingRevoke:NO];
}
/**
* Grant or revoke privileges for the supplied user.
*/
- (BOOL)grantPrivilegesToUser:(SPUserMO *)user skippingRevoke:(BOOL)skipRevoke
{
if ([user valueForKey:@"parent"] != nil)
{
NSMutableArray *grantPrivileges = [NSMutableArray array];
NSMutableArray *revokePrivileges = [NSMutableArray array];
NSArray *changedKeys = [[user changedValues] allKeys];
for (NSString *key in [self privsSupportedByServer])
{
if (![key hasSuffix:@"_priv"]) continue;
//ignore anything that we didn't change
if (![changedKeys containsObject:key]) continue;
NSString *privilege = [key stringByReplacingOccurrencesOfString:@"_priv" withString:@""];
// Check the value of the priv and assign to grant or revoke query as appropriate; do this
// in a try/catch check to avoid exceptions for unhandled privs
NS_DURING
if ([[user valueForKey:key] boolValue] == YES) {
[grantPrivileges addObject:[privilege replaceUnderscoreWithSpace]];
}
else {
[revokePrivileges addObject:[privilege replaceUnderscoreWithSpace]];
}
NS_HANDLER
NS_ENDHANDLER
}
// Grant privileges
if(![self _grantPrivileges:grantPrivileges
onDatabase:nil
forUser:[[user parent] valueForKey:@"user"]
host:[user valueForKey:@"host"]]) return NO;
if(!skipRevoke) {
// Revoke privileges
if(![self _revokePrivileges:revokePrivileges
onDatabase:nil
forUser:[[user parent] valueForKey:@"user"]
host:[user valueForKey:@"host"]]) return NO;
}
}
for (SPPrivilegesMO *priv in [user valueForKey:@"schema_privileges"])
{
if(![self grantDbPrivilegesWithPrivilege:priv skippingRevoke:skipRevoke]) return NO;
}
return YES;
}
#pragma mark -
#pragma mark Private API
/**
* Gets any NSManagedObject (SPUser) from the managedObjectContext that may
* already exist with the given username.
*/
- (NSArray *)_fetchUserWithUserName:(NSString *)username
{
NSManagedObjectContext *moc = [self managedObjectContext];
NSPredicate *predicate = [NSPredicate predicateWithFormat:@"user == %@ AND parent == nil", username];
NSEntityDescription *entityDescription = [NSEntityDescription entityForName:@"SPUser" inManagedObjectContext:moc];
NSFetchRequest *request = [[[NSFetchRequest alloc] init] autorelease];
[request setEntity:entityDescription];
[request setPredicate:predicate];
NSError *error = nil;
NSArray *array = [moc executeFetchRequest:request error:&error];
if (error != nil) {
[NSApp presentError:error];
}
return array;
}
- (NSArray *)_fetchPrivsWithUser:(NSString *)username schema:(NSString *)selectedSchema host:(NSString *)host
{
NSManagedObjectContext *moc = [self managedObjectContext];
NSPredicate *predicate;
NSEntityDescription *privEntity = [NSEntityDescription entityForName:@"Privileges" inManagedObjectContext:moc];
NSFetchRequest *request = [[[NSFetchRequest alloc] init] autorelease];
// Construct the predicate depending on whether a user and schema were supplied;
// blank schemas indicate a default priv value (as per %)
if ([username length]) {
if ([selectedSchema length]) {
predicate = [NSPredicate predicateWithFormat:@"(user.parent.user like[cd] %@) AND (user.host like[cd] %@) AND (db like[cd] %@)", username, host, selectedSchema];
} else {
predicate = [NSPredicate predicateWithFormat:@"(user.parent.user like[cd] %@) AND (user.host like[cd] %@) AND (db == '')", username, host];
}
} else {
if ([selectedSchema length]) {
predicate = [NSPredicate predicateWithFormat:@"(user.parent.user == '') AND (user.host like[cd] %@) AND (db like[cd] %@)", host, selectedSchema];
} else {
predicate = [NSPredicate predicateWithFormat:@"(user.parent.user == '') AND (user.host like[cd] %@) AND (db == '')", host];
}
}
[request setEntity:privEntity];
[request setPredicate:predicate];
NSError *error = nil;
NSArray *array = [moc executeFetchRequest:request error:&error];
if (error != nil) {
[NSApp presentError:error];
}
return array;
}
/**
* Creates a new NSManagedObject and inserts it into the managedObjectContext.
*/
- (SPUserMO *)_createNewSPUser
{
return [NSEntityDescription insertNewObjectForEntityForName:@"SPUser" inManagedObjectContext:[self managedObjectContext]];
}
/**
* Grant the supplied privileges to the specified user and host
*/
- (BOOL)_grantPrivileges:(NSArray *)thePrivileges onDatabase:(NSString *)aDatabase forUser:(NSString *)aUser host:(NSString *)aHost
{
if (![thePrivileges count]) return YES;
NSString *grantStatement;
// Special case when all items are checked, to allow GRANT OPTION to work
if ([[self privsSupportedByServer] count] == [thePrivileges count]) {
grantStatement = [NSString stringWithFormat:@"GRANT ALL ON %@.* TO %@@%@ WITH GRANT OPTION",
aDatabase?[aDatabase backtickQuotedString]:@"*",
[aUser tickQuotedString],
[aHost tickQuotedString]];
}
else {
grantStatement = [NSString stringWithFormat:@"GRANT %@ ON %@.* TO %@@%@",
[[thePrivileges componentsJoinedByCommas] uppercaseString],
aDatabase?[aDatabase backtickQuotedString]:@"*",
[aUser tickQuotedString],
[aHost tickQuotedString]];
}
[connection queryString:grantStatement];
return [self _checkAndDisplayMySqlError];
}
/**
* Revoke the supplied privileges from the specified user and host
*/
- (BOOL)_revokePrivileges:(NSArray *)thePrivileges onDatabase:(NSString *)aDatabase forUser:(NSString *)aUser host:(NSString *)aHost
{
if (![thePrivileges count]) return YES;
NSString *revokeStatement;
// Special case when all items are checked, to allow GRANT OPTION to work
if ([[self privsSupportedByServer] count] == [thePrivileges count]) {
revokeStatement = [NSString stringWithFormat:@"REVOKE ALL PRIVILEGES ON %@.* FROM %@@%@",
aDatabase?[aDatabase backtickQuotedString]:@"*",
[aUser tickQuotedString],
[aHost tickQuotedString]];
[connection queryString:revokeStatement];
if(![self _checkAndDisplayMySqlError]) return NO;
revokeStatement = [NSString stringWithFormat:@"REVOKE GRANT OPTION ON %@.* FROM %@@%@",
aDatabase?[aDatabase backtickQuotedString]:@"*",
[aUser tickQuotedString],
[aHost tickQuotedString]];
}
else {
revokeStatement = [NSString stringWithFormat:@"REVOKE %@ ON %@.* FROM %@@%@",
[[thePrivileges componentsJoinedByCommas] uppercaseString],
aDatabase?[aDatabase backtickQuotedString]:@"*",
[aUser tickQuotedString],
[aHost tickQuotedString]];
}
[connection queryString:revokeStatement];
return [self _checkAndDisplayMySqlError];
}
/**
* Displays an alert panel if there was an error condition on the MySQL connection.
*/
- (BOOL)_checkAndDisplayMySqlError
{
if ([connection queryErrored]) {
if (isSaving) {
[errorsString appendFormat:@"%@\n", [connection lastErrorMessage]];
}
else {
SPOnewayAlertSheet(
NSLocalizedString(@"An error occurred", @"mysql error occurred message"),
[self window],
[NSString stringWithFormat:NSLocalizedString(@"An error occurred whilst trying to perform the operation.\n\nMySQL said: %@", @"mysql error occurred informative message"), [connection lastErrorMessage]]
);
}
return NO;
}
return YES;
}
/**
* Renames a user account using the supplied parameters.
*
* @param originalUser The user's original user name
* @param originalHost The user's original host
* @param newUser The user's new user name
* @param newHost The user's new host
*/
- (BOOL)_renameUserFrom:(NSString *)originalUser host:(NSString *)originalHost to:(NSString *)newUser host:(NSString *)newHost
{
NSString *renameQuery = nil;
if ([serverSupport supportsRenameUser]) {
renameQuery = [NSString stringWithFormat:@"RENAME USER %@@%@ TO %@@%@",
[originalUser tickQuotedString],
[originalHost tickQuotedString],
[newUser tickQuotedString],
[newHost tickQuotedString]];
}
else {
// mysql.user is keyed on user and host so there should only ever be one result,
// but double check before we do the update.
QKQuery *query = [QKQuery selectQueryFromTable:@"user"];
[query setDatabase:SPMySQLDatabase];
[query addField:@"COUNT(1)"];
[query addParameter:@"User" operator:QKEqualityOperator value:originalUser];
[query addParameter:@"Host" operator:QKEqualityOperator value:originalHost];
SPMySQLResult *result = [connection queryString:[query query]];
if ([[[result getRowAsArray] objectAtIndex:0] integerValue] == 1) {
QKQuery *updateQuery = [QKQuery queryTable:@"user"];
[updateQuery setQueryType:QKUpdateQuery];
[updateQuery setDatabase:SPMySQLDatabase];
[updateQuery addFieldToUpdate:@"User" toValue:newUser];
[updateQuery addFieldToUpdate:@"Host" toValue:newHost];
[updateQuery addParameter:@"User" operator:QKEqualityOperator value:originalUser];
[updateQuery addParameter:@"Host" operator:QKEqualityOperator value:originalHost];
renameQuery = [updateQuery query];
}
}
if (renameQuery) {
[connection queryString:renameQuery];
return [self _checkAndDisplayMySqlError];
}
return YES;
}
#pragma mark -
- (void)dealloc
{
[[NSNotificationCenter defaultCenter] removeObserver:self];
SPClear(managedObjectContext);
SPClear(persistentStoreCoordinator);
SPClear(managedObjectModel);
SPClear(privColumnToGrantMap);
SPClear(connection);
SPClear(privsSupportedByServer);
SPClear(schemas);
SPClear(availablePrivs);
SPClear(grantedSchemaPrivs);
SPClear(treeSortDescriptor);
SPClear(treeSortDescriptors);
SPClear(serverSupport);
[super dealloc];
}
@end
| {
"content_hash": "7a916631e59f2e951cf0f4ef4e017b33",
"timestamp": "",
"source": "github",
"line_count": 1545,
"max_line_length": 205,
"avg_line_length": 33.79676375404531,
"alnum_prop": 0.7148000612838976,
"repo_name": "abhibeckert/sequelpro",
"id": "c06025d24eb9a05f7e62a9bc119e453922301753",
"size": "52216",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "Source/SPUserManager.m",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "C",
"bytes": "212677"
},
{
"name": "HTML",
"bytes": "14864"
},
{
"name": "Lex",
"bytes": "18203"
},
{
"name": "Makefile",
"bytes": "2014"
},
{
"name": "Objective-C",
"bytes": "5235910"
},
{
"name": "PLpgSQL",
"bytes": "1454"
},
{
"name": "Perl",
"bytes": "10934"
},
{
"name": "Shell",
"bytes": "44764"
}
],
"symlink_target": ""
} |
<?php
namespace Drupal\system\Plugin\Block;
use Drupal\Core\Block\BlockBase;
use Drupal\Core\Block\MessagesBlockPluginInterface;
use Drupal\Core\Cache\Cache;
/**
* Provides a block to display the messages.
*
* @see drupal_set_message()
*
* @Block(
* id = "system_messages_block",
* admin_label = @Translation("Messages")
* )
*/
class SystemMessagesBlock extends BlockBase implements MessagesBlockPluginInterface {
/**
* {@inheritdoc}
*/
public function defaultConfiguration() {
return array(
'label_display' => FALSE,
);
}
/**
* {@inheritdoc}
*/
public function build() {
return ['#type' => 'status_messages'];
}
/**
* {@inheritdoc}
*/
public function getCacheMaxAge() {
// The messages are session-specific and hence aren't cacheable, but the
// block itself *is* cacheable because it uses a #lazy_builder callback and
// hence the block has a globally cacheable render array.
return Cache::PERMANENT;
}
}
| {
"content_hash": "3d784162e0487af1a1710fd7dcaeae49",
"timestamp": "",
"source": "github",
"line_count": 47,
"max_line_length": 85,
"avg_line_length": 21.21276595744681,
"alnum_prop": 0.6619859578736209,
"repo_name": "aakb/drupalcampaarhus",
"id": "bbfb03695cbdbbefd8c9c7ac66ca7ecbd189a4d1",
"size": "997",
"binary": false,
"copies": "282",
"ref": "refs/heads/develop",
"path": "core/modules/system/src/Plugin/Block/SystemMessagesBlock.php",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "ApacheConf",
"bytes": "8550"
},
{
"name": "CSS",
"bytes": "630454"
},
{
"name": "HTML",
"bytes": "796606"
},
{
"name": "JavaScript",
"bytes": "1134117"
},
{
"name": "PHP",
"bytes": "33834716"
},
{
"name": "Shell",
"bytes": "52696"
}
],
"symlink_target": ""
} |
<?php
namespace Luna\CostManagementBundle\Tests\Controller;
use Symfony\Bundle\FrameworkBundle\Test\WebTestCase;
class DefaultControllerTest extends WebTestCase
{
public function testIndex()
{
$client = static::createClient();
$crawler = $client->request('GET', '/hello/Fabien');
$this->assertTrue($crawler->filter('html:contains("Hello Fabien")')->count() > 0);
}
}
| {
"content_hash": "2ba24c65e04b941f38552075bd41ca92",
"timestamp": "",
"source": "github",
"line_count": 17,
"max_line_length": 90,
"avg_line_length": 24,
"alnum_prop": 0.6813725490196079,
"repo_name": "WorldWarIII/Luna",
"id": "d5328b18b50c3436551ee895fa3f491d27b22ac5",
"size": "408",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "src/Luna/CostManagementBundle/Tests/Controller/DefaultControllerTest.php",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "CSS",
"bytes": "804920"
},
{
"name": "JavaScript",
"bytes": "624223"
},
{
"name": "PHP",
"bytes": "93672"
}
],
"symlink_target": ""
} |
'use strict';
report
.factory('LineChart', function($http){
return {
'getConfig':function(URL){
return $http.post(URL)
}
};
}) | {
"content_hash": "bc64bffd19448478637b1a948b11d4c2",
"timestamp": "",
"source": "github",
"line_count": 9,
"max_line_length": 40,
"avg_line_length": 17.555555555555557,
"alnum_prop": 0.5443037974683544,
"repo_name": "mining/frontend",
"id": "0175702aff92feadfb9a97c3a5d71b3f6551e8d7",
"size": "158",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "assets/app/scripts/report/services.js",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "CSS",
"bytes": "29030"
},
{
"name": "HTML",
"bytes": "113896"
},
{
"name": "JavaScript",
"bytes": "85244"
},
{
"name": "Makefile",
"bytes": "38"
}
],
"symlink_target": ""
} |
description: Lists the properties that are available for the context argument when creating rules.
---
# Context Argument Properties in Rules
When creating [Rules](/rules), one of the input arguments is `context`. This is an object containing contextual information of the current authentication transaction, such as user's IP address, application, location, and so forth.
The following properties are available for the `context` object:
* `clientID`: the client id of the application the user is logging in to.
* `clientName`: the name of the application (as defined on the dashboard).
* `clientMetadata`: is an object, whose keys and values are strings, for holding other client properties.
* `connection`: the name of the connection used to authenticate the user (e.g.: `twitter` or `some-google-apps-domain`)
* `connectionStrategy`: the type of connection. For social connection `connectionStrategy` === `connection`. For enterprise connections, the strategy will be `waad` (Windows Azure AD), `ad` (Active Directory/LDAP), `auth0` (database connections), etc.
* `samlConfiguration`: an object that controls the behavior of the SAML and WS-Fed endpoints. Useful for advanced claims mapping and token enrichment (only available for `samlp` and `wsfed` protocol).
* `jwtConfiguration`: an object to configure how Json Web Tokens (JWT) will be generated:
- `lifetimeInSeconds`: expiration of the token.
- `scopes`: predefined scopes values (e.g.: `{ 'images': ['picture', 'logo'] }` this scope value will request access to the picture and logo claims).
* `protocol`: the authentication protocol. Possible values:
- `oidc-basic-profile`: most used, web based login
- `oidc-implicit-profile`: used on mobile devices and single page apps
- `oauth2-resource-owner`: user/password login typically used on database connections
- `oauth2-resource-owner-jwt-bearer`: login using a bearer JWT signed with user's private key
- `oauth2-password`: login using the password exchange
- `oauth2-refresh-token`: refreshing a token using the refresh token exchange
- `samlp`: SAML protocol used on SaaS apps
- `wsfed`: WS-Federation used on Microsoft products like Office365
- `wstrust-usernamemixed`: WS-trust user/password login used on CRM and Office365
- `delegation`: when calling the [Delegation endpoint](/api/authentication#delegation)
- `redirect-callback`: when a redirect rule is resumed
* `stats`: an object containing specific user stats, like `stats.loginsCount`.
* `sso`: this object will contain information about the SSO transaction (if available)
- `with_auth0`: when a user signs in with SSO to an application where the `Use Auth0 instead of the IdP to do Single Sign On` setting is enabled.
- `with_dbconn`: an SSO login for a user that logged in through a database connection.
- `current_clients`: client IDs using SSO.
* `accessToken`: used to add custom namespaced claims to the `access_token`.
* `idToken`: used to add custom namespaced claims to the `id_token`.
* `sessionID`: unique id for the authentication session.
* `request`: an object containing useful information of the request. It has the following properties:
- `userAgent`: the user-agent of the client that is trying to log in.
- `ip`: the originating IP address of the user trying to log in.
- `hostname`: the hostname that is being used for the authentication flow.
- `query`: an object containing the querystring properties of the login transaction sent by the application.
- `body`: the body of the POST request on login transactions used on `oauth2-resource-owner`, `oauth2-resource-owner-jwt-bearer` or `wstrust-usernamemixed` protocols.
- `geoip`: an object containing geographic IP information. It has the following properties:
- `country_code`: a two-character code for the country associated with the IP address
- `country_code3`: a three-character code for the country associated with the IP address
- `country_name`: the country name associated with the IP address
- `city_name`: the city or town name associated with the IP address
- `latitude`: the latitude associated with the IP address.
- `longitude`: the longitude associated with the IP address.
- `time_zone`: the timezone associated with the IP address.
- `continent_code`: a two-character code for the continent associated with the IP address.
## Sample Object
```js
{
clientID: 'q2hn...pXmTUA',
clientName: 'Default App',
clientMetadata: {},
connection: 'Username-Password-Authentication',
connectionStrategy: 'auth0',
samlConfiguration: {},
jwtConfiguration: {},
protocol: 'oidc-basic-profile',
stats: { loginsCount: 111 },
sso: { with_auth0: false, with_dbconn: false, current_clients: [] },
accessToken: {},
idToken: {},
sessionID: 'jYA5wG...BNT5Bak',
request:
{
userAgent: 'Mozilla/5.0 (Macintosh; Intel Mac OS X 10_12_3) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/56.0.2924.87 Safari/537.36',
ip: '188.6.125.49',
hostname: 'mydomain.auth0.com',
query:
{
scope: 'openid',
response_type: 'code',
connection: 'Username-Password-Authentication',
sso: 'true',
protocol: 'oauth2',
audience: 'my-api',
state: 'nB7rfBCL41nppFxqLQ-3cO75XO1QRFyD',
client_id: 'q2hn...pXmTUA',
redirect_uri: 'http://localhost/callback',
device: 'Browser'
},
body: {},
geoip:
{
country_code: 'GR',
country_code3: 'GRC',
country_name: 'Greece',
city_name: 'Athens',
latitude: 136.9733,
longitude: 125.7233,
time_zone: 'Europe/Athens',
continent_code: 'EU'
}
}
}
```
| {
"content_hash": "c1688a2737d71b09afa343548e71cd59",
"timestamp": "",
"source": "github",
"line_count": 104,
"max_line_length": 251,
"avg_line_length": 54.27884615384615,
"alnum_prop": 0.7215234720992029,
"repo_name": "khorne3/docs",
"id": "04bc457152f26117c2f0f074769876a715d13853",
"size": "5649",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "articles/rules/context.md",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "HTML",
"bytes": "50844"
},
{
"name": "JavaScript",
"bytes": "28697"
},
{
"name": "Shell",
"bytes": "1549"
}
],
"symlink_target": ""
} |
// Copyright 2017 The Oppia Authors. All Rights Reserved.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS-IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
/**
* @fileoverview Component for the solution viewer and editor section in the
* state editor.
*/
require(
'components/common-layout-directives/common-elements/' +
'confirm-or-cancel-modal.controller.ts');
require(
'components/state-directives/response-header/response-header.component.ts');
require(
'components/state-directives/solution-editor/solution-editor.component.ts');
require(
'pages/exploration-editor-page/editor-tab/templates/modal-templates/' +
'add-or-update-solution-modal.controller.ts');
require('domain/exploration/SolutionObjectFactory.ts');
require('domain/utilities/url-interpolation.service.ts');
require('filters/string-utility-filters/convert-to-plain-text.filter.ts');
require(
'pages/exploration-editor-page/editor-tab/services/responses.service.ts');
require(
'pages/exploration-editor-page/editor-tab/services/' +
'solution-validity.service.ts');
require(
'pages/exploration-editor-page/editor-tab/services/' +
'solution-verification.service.ts');
require(
'pages/exploration-player-page/services/current-interaction.service.ts');
require(
'components/state-editor/state-editor-properties-services/' +
'state-customization-args.service.ts');
require(
'components/state-editor/state-editor-properties-services/' +
'state-editor.service.ts');
require(
'components/state-editor/state-editor-properties-services/' +
'state-hints.service.ts');
require(
'components/state-editor/state-editor-properties-services/' +
'state-interaction-id.service.ts');
require(
'components/state-editor/state-editor-properties-services/' +
'state-solution.service.ts');
require(
'pages/exploration-editor-page/editor-tab/services/' +
'solution-verification.service');
require('services/alerts.service.ts');
require('services/editability.service.ts');
require('services/exploration-html-formatter.service.ts');
require('components/state-editor/state-editor.constants.ajs.ts');
require('services/contextual/window-dimensions.service');
require('services/external-save.service.ts');
angular.module('oppia').component('stateSolutionEditor', {
bindings: {
onSaveSolution: '=',
refreshWarnings: '&',
showMarkAllAudioAsNeedingUpdateModalIfRequired: '='
},
template: require('./state-solution-editor.component.html'),
controller: [
'$filter', '$scope', '$uibModal', 'AlertsService', 'EditabilityService',
'ExplorationHtmlFormatterService', 'ExternalSaveService',
'SolutionValidityService', 'SolutionVerificationService',
'StateCustomizationArgsService', 'StateEditorService',
'StateHintsService', 'StateInteractionIdService',
'StateSolutionService',
'WindowDimensionsService',
'INFO_MESSAGE_SOLUTION_IS_INVALID_FOR_EXPLORATION',
'INFO_MESSAGE_SOLUTION_IS_INVALID_FOR_QUESTION',
'INTERACTION_SPECS',
function(
$filter, $scope, $uibModal, AlertsService, EditabilityService,
ExplorationHtmlFormatterService, ExternalSaveService,
SolutionValidityService, SolutionVerificationService,
StateCustomizationArgsService, StateEditorService,
StateHintsService, StateInteractionIdService,
StateSolutionService,
WindowDimensionsService,
INFO_MESSAGE_SOLUTION_IS_INVALID_FOR_EXPLORATION,
INFO_MESSAGE_SOLUTION_IS_INVALID_FOR_QUESTION,
INTERACTION_SPECS) {
var ctrl = this;
$scope.getInvalidSolutionTooltip = function() {
if (StateEditorService.isInQuestionMode()) {
return 'This solution doesn\'t correspond to an answer ' +
'marked as correct. Verify the rules specified for the ' +
'answers or change the solution.';
}
return 'This solution does not lead to another card. Verify the ' +
'responses specified or change the solution.';
};
$scope.isSolutionValid = function() {
return StateEditorService.isCurrentSolutionValid();
};
$scope.toggleInlineSolutionEditorIsActive = function() {
$scope.inlineSolutionEditorIsActive = (
!$scope.inlineSolutionEditorIsActive);
};
$scope.getSolutionSummary = function() {
var solution = StateSolutionService.savedMemento;
var solutionAsPlainText =
solution.getSummary(StateInteractionIdService.savedMemento);
solutionAsPlainText =
$filter('convertToPlainText')(solutionAsPlainText);
return solutionAsPlainText;
};
// This returns false if the current interaction ID is null.
$scope.isCurrentInteractionLinear = function() {
return (
StateInteractionIdService.savedMemento &&
INTERACTION_SPECS[
StateInteractionIdService.savedMemento
].is_linear);
};
$scope.openAddOrUpdateSolutionModal = function() {
AlertsService.clearWarnings();
ExternalSaveService.onExternalSave.emit();
$scope.inlineSolutionEditorIsActive = false;
$uibModal.open({
template: require(
'pages/exploration-editor-page/editor-tab/templates/' +
'modal-templates/add-or-update-solution-modal.template.html'),
backdrop: 'static',
controller: 'AddOrUpdateSolutionModalController'
}).result.then(function(result) {
StateSolutionService.displayed = result.solution;
StateSolutionService.saveDisplayedValue();
ctrl.onSaveSolution(StateSolutionService.displayed);
let solutionIsValid = SolutionVerificationService.verifySolution(
StateEditorService.getActiveStateName(),
StateEditorService.getInteraction(),
StateSolutionService.savedMemento.correctAnswer
);
SolutionValidityService.updateValidity(
StateEditorService.getActiveStateName(), solutionIsValid);
ctrl.refreshWarnings()();
if (!solutionIsValid) {
if (StateEditorService.isInQuestionMode()) {
AlertsService.addInfoMessage(
INFO_MESSAGE_SOLUTION_IS_INVALID_FOR_QUESTION, 4000);
} else {
AlertsService.addInfoMessage(
INFO_MESSAGE_SOLUTION_IS_INVALID_FOR_EXPLORATION, 4000);
}
}
}, function() {
AlertsService.clearWarnings();
});
};
$scope.deleteSolution = function(index, evt) {
evt.stopPropagation();
AlertsService.clearWarnings();
$uibModal.open({
template: require(
'pages/exploration-editor-page/editor-tab/templates/' +
'modal-templates/delete-solution-modal.template.html'),
backdrop: true,
controller: 'ConfirmOrCancelModalController'
}).result.then(function() {
StateSolutionService.displayed = null;
StateSolutionService.saveDisplayedValue();
ctrl.onSaveSolution(StateSolutionService.displayed);
StateEditorService.deleteCurrentSolutionValidity();
ctrl.refreshWarnings()();
}, function() {
AlertsService.clearWarnings();
});
};
$scope.toggleSolutionCard = function() {
$scope.solutionCardIsShown = !$scope.solutionCardIsShown;
};
ctrl.$onInit = function() {
$scope.EditabilityService = EditabilityService;
$scope.solutionCardIsShown = (
!WindowDimensionsService.isWindowNarrow());
$scope.correctAnswer = null;
$scope.inlineSolutionEditorIsActive = false;
$scope.SOLUTION_EDITOR_FOCUS_LABEL = (
'currentCorrectAnswerEditorHtmlForSolutionEditor');
$scope.StateHintsService = StateHintsService;
$scope.StateInteractionIdService = StateInteractionIdService;
$scope.StateSolutionService = StateSolutionService;
StateEditorService.updateStateSolutionEditorInitialised();
$scope.correctAnswerEditorHtml = (
ExplorationHtmlFormatterService.getInteractionHtml(
StateInteractionIdService.savedMemento,
StateCustomizationArgsService.savedMemento,
false,
$scope.SOLUTION_EDITOR_FOCUS_LABEL, null));
};
}
]
});
| {
"content_hash": "3a7dbdb7422629b374dbdd871647cab2",
"timestamp": "",
"source": "github",
"line_count": 220,
"max_line_length": 78,
"avg_line_length": 39.91818181818182,
"alnum_prop": 0.6924390799362332,
"repo_name": "kevinlee12/oppia",
"id": "1385a9f8c718afcaf63706aa69dd264cf12e1ea4",
"size": "8782",
"binary": false,
"copies": "1",
"ref": "refs/heads/develop",
"path": "core/templates/components/state-editor/state-solution-editor/state-solution-editor.component.ts",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "CSS",
"bytes": "205771"
},
{
"name": "HTML",
"bytes": "1835761"
},
{
"name": "JavaScript",
"bytes": "1182599"
},
{
"name": "PEG.js",
"bytes": "71377"
},
{
"name": "Python",
"bytes": "13670639"
},
{
"name": "Shell",
"bytes": "2239"
},
{
"name": "TypeScript",
"bytes": "13024194"
}
],
"symlink_target": ""
} |
<!-- THIS IS AUTO-GENERATED CONTENT. DO NOT MANUALLY EDIT. -->
This image is part of the [balena.io][balena] base image series for IoT devices. The image is optimized for use with [balena.io][balena] and [balenaOS][balena-os], but can be used in any Docker environment running on the appropriate architecture.
.
Some notable features in `balenalib` base images:
- Helpful package installer script called `install_packages` that abstracts away the specifics of the underlying package managers. It will install the named packages with smallest number of dependencies (ignore optional dependencies), clean up the package manager medata and retry if package install fails.
- Working with dynamically plugged devices: each `balenalib` base image has a default `ENTRYPOINT` which is defined as `ENTRYPOINT ["/usr/bin/entry.sh"]`, it checks if the `UDEV` flag is set to true or not (by adding `ENV UDEV=1`) and if true, it will start `udevd` daemon and the relevant device nodes in the container /dev will appear.
For more details, please check the [features overview](https://www.balena.io/docs/reference/base-images/base-images/#features-overview) in our documentation.
# [Image Variants][variants]
The `balenalib` images come in many flavors, each designed for a specific use case.
## `:<version>` or `:<version>-run`
This is the defacto image. The `run` variant is designed to be a slim and minimal variant with only runtime essentials packaged into it.
## `:<version>-build`
The build variant is a heavier image that includes many of the tools required for building from source. This reduces the number of packages that you will need to manually install in your Dockerfile, thus reducing the overall size of all images on your system.
[variants]: https://www.balena.io/docs/reference/base-images/base-images/#run-vs-build?ref=dockerhub
# How to use this image with Balena
This [guide][getting-started] can help you get started with using this base image with balena, there are also some cool [example projects][example-projects] that will give you an idea what can be done with balena.
# What is Go?
Go (a.k.a., Golang) is a programming language first developed at Google. It is a statically-typed language with syntax loosely derived from C, but with additional features such as garbage collection, type safety, some dynamic-typing capabilities, additional built-in types (e.g., variable-length arrays and key-value maps), and a large standard library.
> [wikipedia.org/wiki/Go_(programming_language)](http://en.wikipedia.org/wiki/Go_%28programming_language%29)
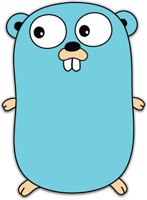
# Supported versions and respective `Dockerfile` links :
[`1.6 (latest)`, `1.15.8`, `1.14.14`](https://github.com/balena-io-library/base-images/tree/master/balena-base-images/golang/raspberrypi4-64/alpine/)
For more information about this image and its history, please see the [relevant manifest file (`raspberrypi4-64-alpine-golang`)](https://github.com/balena-io-library/official-images/blob/master/library/raspberrypi4-64-alpine-golang) in the [`balena-io-library/official-images` GitHub repo](https://github.com/balena-io-library/official-images).
# How to use this image
## Start a Go instance in your app
The most straightforward way to use this image is to use a Go container as both the build and runtime environment. In your `Dockerfile`, writing something along the lines of the following will compile and run your project:
```dockerfile
FROM balenalib/raspberrypi4-64-alpine-golang:latest
WORKDIR /go/src/app
COPY . .
RUN go get -d -v ./...
RUN go install -v ./...
CMD ["app"]
```
You can then build and run the Docker image:
```console
$ docker build -t my-golang-app .
$ docker run -it --rm --name my-running-app my-golang-app
```
## Compile your app inside the Docker container
There may be occasions where it is not appropriate to run your app inside a container. To compile, but not run your app inside the Docker instance, you can write something like:
```console
$ docker run --rm -v "$PWD":/usr/src/myapp -w /usr/src/myapp balenalib/raspberrypi4-64-alpine-golang:latest go build -v
```
This will add your current directory as a volume to the container, set the working directory to the volume, and run the command `go build` which will tell go to compile the project in the working directory and output the executable to `myapp`.
[example-projects]: https://www.balena.io/docs/learn/getting-started/raspberrypi4-64/go/#example-projects?ref=dockerhub
[getting-started]: https://www.balena.io/docs/learn/getting-started/raspberrypi4-64/go/?ref=dockerhub
# User Feedback
## Issues
If you have any problems with or questions about this image, please contact us through a [GitHub issue](https://github.com/balena-io-library/base-images/issues).
## Contributing
You are invited to contribute new features, fixes, or updates, large or small; we are always thrilled to receive pull requests, and do our best to process them as fast as we can.
Before you start to code, we recommend discussing your plans through a [GitHub issue](https://github.com/balena-io-library/base-images/issues), especially for more ambitious contributions. This gives other contributors a chance to point you in the right direction, give you feedback on your design, and help you find out if someone else is working on the same thing.
## Documentation
Documentation for this image is stored in the [base images documentation][docs]. Check it out for list of all of our base images including many specialised ones for e.g. node, python, go, smaller images, etc.
You can also find more details about new features in `balenalib` base images in this [blog post][migration-docs]
[docs]: https://www.balena.io/docs/reference/base-images/base-images/#balena-base-images?ref=dockerhub
[variants]: https://www.balena.io/docs/reference/base-images/base-images/#run-vs-build?ref=dockerhub
[migration-docs]: https://www.balena.io/blog/new-year-new-balena-base-images/?ref=dockerhub
[balena]: https://balena.io/?ref=dockerhub
[balena-os]: https://www.balena.io/os/?ref=dockerhub | {
"content_hash": "aa063e84a5d7d716b2385dc7c97ef9ba",
"timestamp": "",
"source": "github",
"line_count": 107,
"max_line_length": 366,
"avg_line_length": 58.700934579439256,
"alnum_prop": 0.7705779334500875,
"repo_name": "nghiant2710/base-images",
"id": "31b8f701dc58be6775b194679b12032fe50417f5",
"size": "6281",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "balena-base-images/docs/dockerhub/raspberrypi4-64-alpine-golang-full-description.md",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "Dockerfile",
"bytes": "144558581"
},
{
"name": "JavaScript",
"bytes": "16316"
},
{
"name": "Shell",
"bytes": "368690"
}
],
"symlink_target": ""
} |
#import <Foundation/Foundation.h>
#if defined(__cplusplus)
extern "C"
{
#endif /* defined(__cplusplus) */
@interface OLException : NSException
@end /* interface OLException */
@interface OLLibrary : NSObject
+ (BOOL) initialize;
+ (void) uninitialize;
+ (NSString*) getVersionString;
+ (NSUInteger) getVersionMajor;
+ (NSUInteger) getVersionMinor;
+ (NSUInteger) getVersionPatch;
+ (NSString*) getVersionExtra;
@end /* interface OLLibrary */
@interface OLAddress : NSObject
- (instancetype) initWithStreetNum: (int) streetNum
street: (NSString*) street
city: (NSString*) city
province: (NSString* )province
zipCode: (NSString*) zipCode
country: (NSString*) country;
- (void) dealloc;
@property (readonly) int streetNum;
@property (readonly) NSString* street;
@property (readonly) NSString* city;
@property (readonly) NSString* province;
@property (readonly) NSString* zipCode;
@property (readonly) NSString* country;
- (NSString*) toString;
@end /* interface OLAddress */
@interface OLPerson : NSObject
- (instancetype) initWithLastName: (NSString*) lastName
firstName: (NSString*) firstName
age: (int) age
address: (OLAddress*) address;
- (void) dealloc;
@property (readonly) NSString* lastName;
@property (readonly) NSString* firstName;
@property (readonly) int age;
@property (readonly) OLAddress* address;
- (NSString*) toString;
@end /* interface OLPerson */
@protocol OLGenerator <NSObject>
@required
- (void) dealloc;
- (int) generateIntWithData: (int) data;
- (NSString*) generateStringWithData: (int) data;
@end /* protocol OLGenerator */
@interface OLPrinter : NSObject
- (instancetype) initWithGenerator: (id<OLGenerator>) generator;
- (void) dealloc;
- (void) printInt;
- (void) printString;
@end /* interface OLPrinter */
#if defined(__cplusplus)
}
/* extern "C" */
#endif /* defined(__cplusplus) */
| {
"content_hash": "2784088321fc762fb995e89eedfc0758",
"timestamp": "",
"source": "github",
"line_count": 84,
"max_line_length": 64,
"avg_line_length": 22.25,
"alnum_prop": 0.7137506688068486,
"repo_name": "vycasas/language.bindings",
"id": "a217a4d926e4283714c45a4f4cb825e12e22d07d",
"size": "1869",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "objc/code/headers/dotslashzero/objclib/ObjCLib.h",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "C",
"bytes": "178976"
},
{
"name": "C#",
"bytes": "47336"
},
{
"name": "C++",
"bytes": "59423"
},
{
"name": "CMake",
"bytes": "44304"
},
{
"name": "Java",
"bytes": "17381"
},
{
"name": "JavaScript",
"bytes": "7452"
},
{
"name": "Objective-C",
"bytes": "19067"
},
{
"name": "Python",
"bytes": "10695"
},
{
"name": "Swift",
"bytes": "18077"
}
],
"symlink_target": ""
} |
define(['square/directives/directives'], function (directives) {
'use strict';
directives.directive('squareInputDigits', function () {
return {
restrict: 'A',
require: 'ngModel',
link: function (scope, element, attr, ctrl) {
element.setMask({mask: '9', type: 'repeat', autoTab: false});
ctrl.$formatters.push(function (value) {
var validated = !value || /^\d+$/.test(value);
if (validated) {
element.removeAttr('data-square-validate-digits');
} else {
element.attr('data-square-validate-digits', scope.$eval(attr.squareInputDigitsMessage) || I18n.t('square.validation.type.digits'));
}
ctrl.$setValidity('digits', validated);
return value;
});
ctrl.$parsers.push(function (value) {
if (!value || /^\d+$/.test(value)) {
element.removeAttr('data-square-validate-digits');
ctrl.$setValidity('digits', true);
return value ? Number(value) : null;
} else {
element.attr('data-square-validate-digits', scope.$eval(attr.squareInputDigitsMessage) || I18n.t('square.validation.type.digits'));
ctrl.$setValidity('digits', false);
return undefined;
}
});
}
};
});
}); | {
"content_hash": "7bf995e4d20952ab195df353edef0e03",
"timestamp": "",
"source": "github",
"line_count": 43,
"max_line_length": 137,
"avg_line_length": 28.3953488372093,
"alnum_prop": 0.6126126126126126,
"repo_name": "leonardosalles/squarespa",
"id": "3ba6e614ad3b11772b91cc69c179a296f73ab3bf",
"size": "1221",
"binary": false,
"copies": "2",
"ref": "refs/heads/master",
"path": "squareframework-ui/src/main/resources/js/directives/inputDigits.js",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "CSS",
"bytes": "98946"
},
{
"name": "Java",
"bytes": "33118"
},
{
"name": "JavaScript",
"bytes": "319352"
}
],
"symlink_target": ""
} |
using System.Net.Http;
using System.Security.Claims;
using System.Threading.Tasks;
using DevZH.AspNetCore.Authentication.Internal;
using DevZH.AspNetCore.Builder;
using Microsoft.AspNetCore.Authentication;
using Microsoft.AspNetCore.Authentication.OAuth;
using Microsoft.AspNetCore.Http.Authentication;
using Microsoft.AspNetCore.Http.Extensions;
using Newtonsoft.Json.Linq;
namespace DevZH.AspNetCore.Authentication.Qihoo
{
/// <summary>
/// 对一系列认证过程的调控
/// </summary>
public class QihooHandler : OAuthHandler<QihooOptions>
{
public QihooHandler(HttpClient backchannel) : base(backchannel)
{
}
/// <summary>
/// 主要生成的是 Authorization 的链接。
/// </summary>
protected override string BuildChallengeUrl(AuthenticationProperties properties, string redirectUri)
{
var queryBuilder = new QueryBuilder {
{ "client_id", Options.ClientId },
{ "scope", FormatScope() },
{ "response_type", "code" },
{ "redirect_uri", redirectUri },
{ "state", Options.StateDataFormat.Protect(properties) },
{ "display", Options.Display.GetDescription() },
{ "relogin", Options.ReLogin }
};
return Options.AuthorizationEndpoint + queryBuilder;
}
/// <summary>
/// 根据获取到的 token,来得到登录用户的基本信息,并配对。
/// </summary>
protected override async Task<AuthenticationTicket> CreateTicketAsync(ClaimsIdentity identity, AuthenticationProperties properties, OAuthTokenResponse tokens)
{
var endpoint = Options.UserInformationEndpoint + "?access_token=" + UrlEncoder.Encode(tokens.AccessToken);
var response = await Backchannel.GetAsync(endpoint, Context.RequestAborted);
if (!response.IsSuccessStatusCode)
{
throw new HttpRequestException($"Failed to retrieve Qihoo user information ({response.StatusCode}) Please check if the authentication information is correct and the corresponding Qihoo API is enabled.");
}
var payload = JObject.Parse(await response.Content.ReadAsStringAsync());
var ticket = new AuthenticationTicket(new ClaimsPrincipal(identity), properties, Options.AuthenticationScheme);
var context = new OAuthCreatingTicketContext(ticket, Context, Options, Backchannel, tokens, payload);
var identifier = QihooHelper.GetId(payload);
if (!string.IsNullOrEmpty(identifier))
{
identity.AddClaim(new Claim(ClaimTypes.NameIdentifier, identifier, ClaimValueTypes.String, Options.ClaimsIssuer));
identity.AddClaim(new Claim("urn:qihoo:id", identifier, ClaimValueTypes.String, Options.ClaimsIssuer));
}
var name = QihooHelper.GetName(payload);
if (!string.IsNullOrEmpty(name))
{
identity.AddClaim(new Claim(ClaimTypes.Name, name, ClaimValueTypes.String, Options.ClaimsIssuer));
identity.AddClaim(new Claim("urn:qihoo:name", name, ClaimValueTypes.String, Options.ClaimsIssuer));
}
var avatar = QihooHelper.GetAvatar(payload);
if (!string.IsNullOrEmpty(avatar))
{
identity.AddClaim(new Claim("urn:qihoo:avatar", avatar, ClaimValueTypes.String, Options.ClaimsIssuer));
}
await Options.Events.CreatingTicket(context);
return context.Ticket;
}
}
}
| {
"content_hash": "c5ae3e2cf60bcdde0964ad9fd422c003",
"timestamp": "",
"source": "github",
"line_count": 83,
"max_line_length": 219,
"avg_line_length": 42.963855421686745,
"alnum_prop": 0.6475042063937184,
"repo_name": "noliar/MoreAuthentication",
"id": "0bf060f96bcd2b27b709fd587210d9c888887ed2",
"size": "3658",
"binary": false,
"copies": "1",
"ref": "refs/heads/dev",
"path": "src/DevZH.AspNetCore.Authentication.Qihoo/QihooHandler.cs",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "C#",
"bytes": "144360"
}
],
"symlink_target": ""
} |
.class public Landroid/webkit/WebView;
.super Landroid/widget/AbsoluteLayout;
.source "WebView.java"
# interfaces
.implements Landroid/view/ViewTreeObserver$OnGlobalFocusChangeListener;
.implements Landroid/view/ViewGroup$OnHierarchyChangeListener;
.implements Landroid/view/ViewDebug$HierarchyHandler;
# annotations
.annotation system Ldalvik/annotation/MemberClasses;
value = {
Landroid/webkit/WebView$PrivateAccess;,
Landroid/webkit/WebView$HitTestResult;,
Landroid/webkit/WebView$PictureListener;,
Landroid/webkit/WebView$FindListener;,
Landroid/webkit/WebView$WebViewTransport;
}
.end annotation
# static fields
.field private static final LOGTAG:Ljava/lang/String; = "webview_proxy"
.field public static final SCHEME_GEO:Ljava/lang/String; = "geo:0,0?q="
.field public static final SCHEME_MAILTO:Ljava/lang/String; = "mailto:"
.field public static final SCHEME_TEL:Ljava/lang/String; = "tel:"
# instance fields
.field private mProvider:Landroid/webkit/WebViewProvider;
# direct methods
.method public constructor <init>(Landroid/content/Context;)V
.locals 1
.parameter "context"
.prologue
.line 440
const/4 v0, 0x0
invoke-direct {p0, p1, v0}, Landroid/webkit/WebView;-><init>(Landroid/content/Context;Landroid/util/AttributeSet;)V
.line 441
return-void
.end method
.method public constructor <init>(Landroid/content/Context;Landroid/util/AttributeSet;)V
.locals 1
.parameter "context"
.parameter "attrs"
.prologue
.line 450
const v0, #attr@webViewStyle#t
invoke-direct {p0, p1, p2, v0}, Landroid/webkit/WebView;-><init>(Landroid/content/Context;Landroid/util/AttributeSet;I)V
.line 451
return-void
.end method
.method public constructor <init>(Landroid/content/Context;Landroid/util/AttributeSet;I)V
.locals 1
.parameter "context"
.parameter "attrs"
.parameter "defStyle"
.prologue
.line 461
const/4 v0, 0x0
invoke-direct {p0, p1, p2, p3, v0}, Landroid/webkit/WebView;-><init>(Landroid/content/Context;Landroid/util/AttributeSet;IZ)V
.line 462
return-void
.end method
.method protected constructor <init>(Landroid/content/Context;Landroid/util/AttributeSet;ILjava/util/Map;Z)V
.locals 2
.parameter "context"
.parameter "attrs"
.parameter "defStyle"
.parameter
.parameter "privateBrowsing"
.annotation system Ldalvik/annotation/Signature;
value = {
"(",
"Landroid/content/Context;",
"Landroid/util/AttributeSet;",
"I",
"Ljava/util/Map",
"<",
"Ljava/lang/String;",
"Ljava/lang/Object;",
">;Z)V"
}
.end annotation
.prologue
.line 504
.local p4, javaScriptInterfaces:Ljava/util/Map;,"Ljava/util/Map<Ljava/lang/String;Ljava/lang/Object;>;"
invoke-direct {p0, p1, p2, p3}, Landroid/widget/AbsoluteLayout;-><init>(Landroid/content/Context;Landroid/util/AttributeSet;I)V
.line 505
if-nez p1, :cond_0
.line 506
new-instance v0, Ljava/lang/IllegalArgumentException;
const-string v1, "Invalid context argument"
invoke-direct {v0, v1}, Ljava/lang/IllegalArgumentException;-><init>(Ljava/lang/String;)V
throw v0
.line 508
:cond_0
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 510
invoke-direct {p0}, Landroid/webkit/WebView;->ensureProviderCreated()V
.line 511
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p4, p5}, Landroid/webkit/WebViewProvider;->init(Ljava/util/Map;Z)V
.line 512
return-void
.end method
.method public constructor <init>(Landroid/content/Context;Landroid/util/AttributeSet;IZ)V
.locals 6
.parameter "context"
.parameter "attrs"
.parameter "defStyle"
.parameter "privateBrowsing"
.annotation runtime Ljava/lang/Deprecated;
.end annotation
.prologue
.line 481
const/4 v4, 0x0
move-object v0, p0
move-object v1, p1
move-object v2, p2
move v3, p3
move v5, p4
invoke-direct/range {v0 .. v5}, Landroid/webkit/WebView;-><init>(Landroid/content/Context;Landroid/util/AttributeSet;ILjava/util/Map;Z)V
.line 482
return-void
.end method
.method static synthetic access$001(Landroid/webkit/WebView;)I
.locals 1
.parameter "x0"
.prologue
.line 262
invoke-super {p0}, Landroid/view/View;->getScrollBarStyle()I
move-result v0
return v0
.end method
.method static synthetic access$1001(Landroid/webkit/WebView;Landroid/view/ViewGroup$LayoutParams;)V
.locals 0
.parameter "x0"
.parameter "x1"
.prologue
.line 262
invoke-super {p0, p1}, Landroid/view/View;->setLayoutParams(Landroid/view/ViewGroup$LayoutParams;)V
return-void
.end method
.method static synthetic access$101(Landroid/webkit/WebView;II)V
.locals 0
.parameter "x0"
.parameter "x1"
.parameter "x2"
.prologue
.line 262
invoke-super {p0, p1, p2}, Landroid/view/View;->scrollTo(II)V
return-void
.end method
.method static synthetic access$1100(Landroid/webkit/WebView;IIIIIIIIZ)Z
.locals 1
.parameter "x0"
.parameter "x1"
.parameter "x2"
.parameter "x3"
.parameter "x4"
.parameter "x5"
.parameter "x6"
.parameter "x7"
.parameter "x8"
.parameter "x9"
.prologue
.line 262
invoke-virtual/range {p0 .. p9}, Landroid/webkit/WebView;->overScrollBy(IIIIIIIIZ)Z
move-result v0
return v0
.end method
.method static synthetic access$1200(Landroid/webkit/WebView;I)Z
.locals 1
.parameter "x0"
.parameter "x1"
.prologue
.line 262
invoke-virtual {p0, p1}, Landroid/webkit/WebView;->awakenScrollBars(I)Z
move-result v0
return v0
.end method
.method static synthetic access$1300(Landroid/webkit/WebView;IZ)Z
.locals 1
.parameter "x0"
.parameter "x1"
.parameter "x2"
.prologue
.line 262
invoke-virtual {p0, p1, p2}, Landroid/webkit/WebView;->awakenScrollBars(IZ)Z
move-result v0
return v0
.end method
.method static synthetic access$1400(Landroid/webkit/WebView;)F
.locals 1
.parameter "x0"
.prologue
.line 262
invoke-virtual {p0}, Landroid/webkit/WebView;->getVerticalScrollFactor()F
move-result v0
return v0
.end method
.method static synthetic access$1500(Landroid/webkit/WebView;)F
.locals 1
.parameter "x0"
.prologue
.line 262
invoke-virtual {p0}, Landroid/webkit/WebView;->getHorizontalScrollFactor()F
move-result v0
return v0
.end method
.method static synthetic access$1600(Landroid/webkit/WebView;II)V
.locals 0
.parameter "x0"
.parameter "x1"
.parameter "x2"
.prologue
.line 262
invoke-virtual {p0, p1, p2}, Landroid/webkit/WebView;->setMeasuredDimension(II)V
return-void
.end method
.method static synthetic access$1700(Landroid/webkit/WebView;)I
.locals 1
.parameter "x0"
.prologue
.line 262
invoke-virtual {p0}, Landroid/webkit/WebView;->getHorizontalScrollbarHeight()I
move-result v0
return v0
.end method
.method static synthetic access$1802(Landroid/webkit/WebView;I)I
.locals 0
.parameter "x0"
.parameter "x1"
.prologue
.line 262
iput p1, p0, Landroid/webkit/WebView;->mScrollX:I
return p1
.end method
.method static synthetic access$1902(Landroid/webkit/WebView;I)I
.locals 0
.parameter "x0"
.parameter "x1"
.prologue
.line 262
iput p1, p0, Landroid/webkit/WebView;->mScrollY:I
return p1
.end method
.method static synthetic access$201(Landroid/webkit/WebView;)V
.locals 0
.parameter "x0"
.prologue
.line 262
invoke-super {p0}, Landroid/view/View;->computeScroll()V
return-void
.end method
.method static synthetic access$301(Landroid/webkit/WebView;Landroid/view/MotionEvent;)Z
.locals 1
.parameter "x0"
.parameter "x1"
.prologue
.line 262
invoke-super {p0, p1}, Landroid/view/View;->onHoverEvent(Landroid/view/MotionEvent;)Z
move-result v0
return v0
.end method
.method static synthetic access$401(Landroid/webkit/WebView;ILandroid/os/Bundle;)Z
.locals 1
.parameter "x0"
.parameter "x1"
.parameter "x2"
.prologue
.line 262
invoke-super {p0, p1, p2}, Landroid/view/View;->performAccessibilityAction(ILandroid/os/Bundle;)Z
move-result v0
return v0
.end method
.method static synthetic access$501(Landroid/webkit/WebView;)Z
.locals 1
.parameter "x0"
.prologue
.line 262
invoke-super {p0}, Landroid/view/View;->performLongClick()Z
move-result v0
return v0
.end method
.method static synthetic access$601(Landroid/webkit/WebView;IIII)Z
.locals 1
.parameter "x0"
.parameter "x1"
.parameter "x2"
.parameter "x3"
.parameter "x4"
.prologue
.line 262
invoke-super {p0, p1, p2, p3, p4}, Landroid/view/View;->setFrame(IIII)Z
move-result v0
return v0
.end method
.method static synthetic access$701(Landroid/webkit/WebView;Landroid/view/KeyEvent;)Z
.locals 1
.parameter "x0"
.parameter "x1"
.prologue
.line 262
invoke-super {p0, p1}, Landroid/view/ViewGroup;->dispatchKeyEvent(Landroid/view/KeyEvent;)Z
move-result v0
return v0
.end method
.method static synthetic access$801(Landroid/webkit/WebView;Landroid/view/MotionEvent;)Z
.locals 1
.parameter "x0"
.parameter "x1"
.prologue
.line 262
invoke-super {p0, p1}, Landroid/view/View;->onGenericMotionEvent(Landroid/view/MotionEvent;)Z
move-result v0
return v0
.end method
.method static synthetic access$901(Landroid/webkit/WebView;ILandroid/graphics/Rect;)Z
.locals 1
.parameter "x0"
.parameter "x1"
.parameter "x2"
.prologue
.line 262
invoke-super {p0, p1, p2}, Landroid/view/ViewGroup;->requestFocus(ILandroid/graphics/Rect;)Z
move-result v0
return v0
.end method
.method private static checkThread()V
.locals 3
.prologue
.line 1875
invoke-static {}, Landroid/os/Looper;->myLooper()Landroid/os/Looper;
move-result-object v1
invoke-static {}, Landroid/os/Looper;->getMainLooper()Landroid/os/Looper;
move-result-object v2
if-eq v1, v2, :cond_0
.line 1876
new-instance v0, Ljava/lang/Throwable;
new-instance v1, Ljava/lang/StringBuilder;
invoke-direct {v1}, Ljava/lang/StringBuilder;-><init>()V
const-string v2, "Warning: A WebView method was called on thread \'"
invoke-virtual {v1, v2}, Ljava/lang/StringBuilder;->append(Ljava/lang/String;)Ljava/lang/StringBuilder;
move-result-object v1
invoke-static {}, Ljava/lang/Thread;->currentThread()Ljava/lang/Thread;
move-result-object v2
invoke-virtual {v2}, Ljava/lang/Thread;->getName()Ljava/lang/String;
move-result-object v2
invoke-virtual {v1, v2}, Ljava/lang/StringBuilder;->append(Ljava/lang/String;)Ljava/lang/StringBuilder;
move-result-object v1
const-string v2, "\'. "
invoke-virtual {v1, v2}, Ljava/lang/StringBuilder;->append(Ljava/lang/String;)Ljava/lang/StringBuilder;
move-result-object v1
const-string v2, "All WebView methods must be called on the UI thread. "
invoke-virtual {v1, v2}, Ljava/lang/StringBuilder;->append(Ljava/lang/String;)Ljava/lang/StringBuilder;
move-result-object v1
const-string v2, "Future versions of WebView may not support use on other threads."
invoke-virtual {v1, v2}, Ljava/lang/StringBuilder;->append(Ljava/lang/String;)Ljava/lang/StringBuilder;
move-result-object v1
invoke-virtual {v1}, Ljava/lang/StringBuilder;->toString()Ljava/lang/String;
move-result-object v1
invoke-direct {v0, v1}, Ljava/lang/Throwable;-><init>(Ljava/lang/String;)V
.line 1881
.local v0, throwable:Ljava/lang/Throwable;
const-string/jumbo v1, "webview_proxy"
invoke-static {v0}, Landroid/util/Log;->getStackTraceString(Ljava/lang/Throwable;)Ljava/lang/String;
move-result-object v2
invoke-static {v1, v2}, Landroid/util/Log;->w(Ljava/lang/String;Ljava/lang/String;)I
.line 1882
invoke-static {v0}, Landroid/os/StrictMode;->onWebViewMethodCalledOnWrongThread(Ljava/lang/Throwable;)V
.line 1884
:cond_0
return-void
.end method
.method public static disablePlatformNotifications()V
.locals 2
.annotation runtime Ljava/lang/Deprecated;
.end annotation
.prologue
.line 679
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 680
invoke-static {}, Landroid/webkit/WebView;->getFactory()Landroid/webkit/WebViewFactoryProvider;
move-result-object v0
invoke-interface {v0}, Landroid/webkit/WebViewFactoryProvider;->getStatics()Landroid/webkit/WebViewFactoryProvider$Statics;
move-result-object v0
const/4 v1, 0x0
invoke-interface {v0, v1}, Landroid/webkit/WebViewFactoryProvider$Statics;->setPlatformNotificationsEnabled(Z)V
.line 681
return-void
.end method
.method public static enablePlatformNotifications()V
.locals 2
.annotation runtime Ljava/lang/Deprecated;
.end annotation
.prologue
.line 666
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 667
invoke-static {}, Landroid/webkit/WebView;->getFactory()Landroid/webkit/WebViewFactoryProvider;
move-result-object v0
invoke-interface {v0}, Landroid/webkit/WebViewFactoryProvider;->getStatics()Landroid/webkit/WebViewFactoryProvider$Statics;
move-result-object v0
const/4 v1, 0x1
invoke-interface {v0, v1}, Landroid/webkit/WebViewFactoryProvider$Statics;->setPlatformNotificationsEnabled(Z)V
.line 668
return-void
.end method
.method private ensureProviderCreated()V
.locals 2
.prologue
.line 1859
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1860
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
if-nez v0, :cond_0
.line 1863
invoke-static {}, Landroid/webkit/WebView;->getFactory()Landroid/webkit/WebViewFactoryProvider;
move-result-object v0
new-instance v1, Landroid/webkit/WebView$PrivateAccess;
invoke-direct {v1, p0}, Landroid/webkit/WebView$PrivateAccess;-><init>(Landroid/webkit/WebView;)V
invoke-interface {v0, p0, v1}, Landroid/webkit/WebViewFactoryProvider;->createWebView(Landroid/webkit/WebView;Landroid/webkit/WebView$PrivateAccess;)Landroid/webkit/WebViewProvider;
move-result-object v0
iput-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
.line 1865
:cond_0
return-void
.end method
.method public static findAddress(Ljava/lang/String;)Ljava/lang/String;
.locals 1
.parameter "addr"
.prologue
.line 1418
invoke-static {}, Landroid/webkit/WebView;->getFactory()Landroid/webkit/WebViewFactoryProvider;
move-result-object v0
invoke-interface {v0}, Landroid/webkit/WebViewFactoryProvider;->getStatics()Landroid/webkit/WebViewFactoryProvider$Statics;
move-result-object v0
invoke-interface {v0, p0}, Landroid/webkit/WebViewFactoryProvider$Statics;->findAddress(Ljava/lang/String;)Ljava/lang/String;
move-result-object v0
return-object v0
.end method
.method private static declared-synchronized getFactory()Landroid/webkit/WebViewFactoryProvider;
.locals 2
.prologue
.line 1870
const-class v1, Landroid/webkit/WebView;
monitor-enter v1
:try_start_0
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1871
invoke-static {}, Landroid/webkit/WebViewFactory;->getProvider()Landroid/webkit/WebViewFactoryProvider;
:try_end_0
.catchall {:try_start_0 .. :try_end_0} :catchall_0
move-result-object v0
monitor-exit v1
return-object v0
.line 1870
:catchall_0
move-exception v0
monitor-exit v1
throw v0
.end method
.method public static declared-synchronized getPluginList()Landroid/webkit/PluginList;
.locals 2
.annotation runtime Ljava/lang/Deprecated;
.end annotation
.prologue
.line 1572
const-class v1, Landroid/webkit/WebView;
monitor-enter v1
:try_start_0
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1573
new-instance v0, Landroid/webkit/PluginList;
invoke-direct {v0}, Landroid/webkit/PluginList;-><init>()V
:try_end_0
.catchall {:try_start_0 .. :try_end_0} :catchall_0
monitor-exit v1
return-object v0
.line 1572
:catchall_0
move-exception v0
monitor-exit v1
throw v0
.end method
# virtual methods
.method public addJavascriptInterface(Ljava/lang/Object;Ljava/lang/String;)V
.locals 1
.parameter "object"
.parameter "name"
.prologue
.line 1535
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1536
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1, p2}, Landroid/webkit/WebViewProvider;->addJavascriptInterface(Ljava/lang/Object;Ljava/lang/String;)V
.line 1537
return-void
.end method
.method public canGoBack()Z
.locals 1
.prologue
.line 912
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 913
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->canGoBack()Z
move-result v0
return v0
.end method
.method public canGoBackOrForward(I)Z
.locals 1
.parameter "steps"
.prologue
.line 950
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 951
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider;->canGoBackOrForward(I)Z
move-result v0
return v0
.end method
.method public canGoForward()Z
.locals 1
.prologue
.line 930
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 931
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->canGoForward()Z
move-result v0
return v0
.end method
.method public canZoomIn()Z
.locals 1
.annotation runtime Ljava/lang/Deprecated;
.end annotation
.prologue
.line 1669
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1670
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->canZoomIn()Z
move-result v0
return v0
.end method
.method public canZoomOut()Z
.locals 1
.annotation runtime Ljava/lang/Deprecated;
.end annotation
.prologue
.line 1684
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1685
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->canZoomOut()Z
move-result v0
return v0
.end method
.method public capturePicture()Landroid/graphics/Picture;
.locals 1
.prologue
.line 1021
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1022
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->capturePicture()Landroid/graphics/Picture;
move-result-object v0
return-object v0
.end method
.method public clearCache(Z)V
.locals 1
.parameter "includeDiskFiles"
.prologue
.line 1278
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1279
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider;->clearCache(Z)V
.line 1280
return-void
.end method
.method public clearFormData()V
.locals 1
.prologue
.line 1289
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1290
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->clearFormData()V
.line 1291
return-void
.end method
.method public clearHistory()V
.locals 1
.prologue
.line 1297
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1298
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->clearHistory()V
.line 1299
return-void
.end method
.method public clearMatches()V
.locals 1
.prologue
.line 1426
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1427
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->clearMatches()V
.line 1428
return-void
.end method
.method public clearSslPreferences()V
.locals 1
.prologue
.line 1306
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1307
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->clearSslPreferences()V
.line 1308
return-void
.end method
.method public clearView()V
.locals 1
.prologue
.line 1002
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1003
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->clearView()V
.line 1004
return-void
.end method
.method protected computeHorizontalScrollOffset()I
.locals 1
.prologue
.line 1933
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getScrollDelegate()Landroid/webkit/WebViewProvider$ScrollDelegate;
move-result-object v0
invoke-interface {v0}, Landroid/webkit/WebViewProvider$ScrollDelegate;->computeHorizontalScrollOffset()I
move-result v0
return v0
.end method
.method protected computeHorizontalScrollRange()I
.locals 1
.prologue
.line 1928
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getScrollDelegate()Landroid/webkit/WebViewProvider$ScrollDelegate;
move-result-object v0
invoke-interface {v0}, Landroid/webkit/WebViewProvider$ScrollDelegate;->computeHorizontalScrollRange()I
move-result v0
return v0
.end method
.method public computeScroll()V
.locals 1
.prologue
.line 1953
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getScrollDelegate()Landroid/webkit/WebViewProvider$ScrollDelegate;
move-result-object v0
invoke-interface {v0}, Landroid/webkit/WebViewProvider$ScrollDelegate;->computeScroll()V
.line 1954
return-void
.end method
.method protected computeVerticalScrollExtent()I
.locals 1
.prologue
.line 1948
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getScrollDelegate()Landroid/webkit/WebViewProvider$ScrollDelegate;
move-result-object v0
invoke-interface {v0}, Landroid/webkit/WebViewProvider$ScrollDelegate;->computeVerticalScrollExtent()I
move-result v0
return v0
.end method
.method protected computeVerticalScrollOffset()I
.locals 1
.prologue
.line 1943
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getScrollDelegate()Landroid/webkit/WebViewProvider$ScrollDelegate;
move-result-object v0
invoke-interface {v0}, Landroid/webkit/WebViewProvider$ScrollDelegate;->computeVerticalScrollOffset()I
move-result v0
return v0
.end method
.method protected computeVerticalScrollRange()I
.locals 1
.prologue
.line 1938
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getScrollDelegate()Landroid/webkit/WebViewProvider$ScrollDelegate;
move-result-object v0
invoke-interface {v0}, Landroid/webkit/WebViewProvider$ScrollDelegate;->computeVerticalScrollRange()I
move-result v0
return v0
.end method
.method public copyBackForwardList()Landroid/webkit/WebBackForwardList;
.locals 1
.prologue
.line 1319
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1320
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->copyBackForwardList()Landroid/webkit/WebBackForwardList;
move-result-object v0
return-object v0
.end method
.method public debugDump()V
.locals 0
.annotation runtime Ljava/lang/Deprecated;
.end annotation
.prologue
.line 1714
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1715
return-void
.end method
.method public destroy()V
.locals 1
.prologue
.line 653
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 654
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->destroy()V
.line 655
return-void
.end method
.method public dispatchKeyEvent(Landroid/view/KeyEvent;)Z
.locals 1
.parameter "event"
.prologue
.line 2110
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider$ViewDelegate;->dispatchKeyEvent(Landroid/view/KeyEvent;)Z
move-result v0
return v0
.end method
.method public documentHasImages(Landroid/os/Message;)V
.locals 1
.parameter "response"
.prologue
.line 1438
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1439
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider;->documentHasImages(Landroid/os/Message;)V
.line 1440
return-void
.end method
.method public dumpViewHierarchyWithProperties(Ljava/io/BufferedWriter;I)V
.locals 1
.parameter "out"
.parameter "level"
.prologue
.line 1723
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1, p2}, Landroid/webkit/WebViewProvider;->dumpViewHierarchyWithProperties(Ljava/io/BufferedWriter;I)V
.line 1724
return-void
.end method
.method public emulateShiftHeld()V
.locals 0
.annotation runtime Ljava/lang/Deprecated;
.end annotation
.prologue
.line 1594
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1595
return-void
.end method
.method public findAll(Ljava/lang/String;)I
.locals 1
.parameter "find"
.annotation runtime Ljava/lang/Deprecated;
.end annotation
.prologue
.line 1361
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1362
const-string v0, "findAll blocks UI: prefer findAllAsync"
invoke-static {v0}, Landroid/os/StrictMode;->noteSlowCall(Ljava/lang/String;)V
.line 1363
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider;->findAll(Ljava/lang/String;)I
move-result v0
return v0
.end method
.method public findAllAsync(Ljava/lang/String;)V
.locals 1
.parameter "find"
.prologue
.line 1375
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1376
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider;->findAllAsync(Ljava/lang/String;)V
.line 1377
return-void
.end method
.method public findHierarchyView(Ljava/lang/String;I)Landroid/view/View;
.locals 1
.parameter "className"
.parameter "hashCode"
.prologue
.line 1732
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1, p2}, Landroid/webkit/WebViewProvider;->findHierarchyView(Ljava/lang/String;I)Landroid/view/View;
move-result-object v0
return-object v0
.end method
.method public findNext(Z)V
.locals 1
.parameter "forward"
.prologue
.line 1346
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1347
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider;->findNext(Z)V
.line 1348
return-void
.end method
.method public flingScroll(II)V
.locals 1
.parameter "vx"
.parameter "vy"
.prologue
.line 1636
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1637
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1, p2}, Landroid/webkit/WebViewProvider;->flingScroll(II)V
.line 1638
return-void
.end method
.method public freeMemory()V
.locals 1
.prologue
.line 1267
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1268
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->freeMemory()V
.line 1269
return-void
.end method
.method public getCertificate()Landroid/net/http/SslCertificate;
.locals 1
.prologue
.line 572
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 573
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getCertificate()Landroid/net/http/SslCertificate;
move-result-object v0
return-object v0
.end method
.method public getContentHeight()I
.locals 1
.annotation runtime Landroid/view/ViewDebug$ExportedProperty;
category = "webview"
.end annotation
.prologue
.line 1198
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1199
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getContentHeight()I
move-result v0
return v0
.end method
.method public getContentWidth()I
.locals 1
.annotation runtime Landroid/view/ViewDebug$ExportedProperty;
category = "webview"
.end annotation
.prologue
.line 1210
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getContentWidth()I
move-result v0
return v0
.end method
.method public getFavicon()Landroid/graphics/Bitmap;
.locals 1
.prologue
.line 1166
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1167
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getFavicon()Landroid/graphics/Bitmap;
move-result-object v0
return-object v0
.end method
.method public getHitTestResult()Landroid/webkit/WebView$HitTestResult;
.locals 1
.prologue
.line 1085
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1086
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getHitTestResult()Landroid/webkit/WebView$HitTestResult;
move-result-object v0
return-object v0
.end method
.method public getHttpAuthUsernamePassword(Ljava/lang/String;Ljava/lang/String;)[Ljava/lang/String;
.locals 1
.parameter "host"
.parameter "realm"
.prologue
.line 643
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 644
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1, p2}, Landroid/webkit/WebViewProvider;->getHttpAuthUsernamePassword(Ljava/lang/String;Ljava/lang/String;)[Ljava/lang/String;
move-result-object v0
return-object v0
.end method
.method public getOriginalUrl()Ljava/lang/String;
.locals 1
.annotation runtime Landroid/view/ViewDebug$ExportedProperty;
category = "webview"
.end annotation
.prologue
.line 1143
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1144
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getOriginalUrl()Ljava/lang/String;
move-result-object v0
return-object v0
.end method
.method public getProgress()I
.locals 1
.prologue
.line 1187
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1188
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getProgress()I
move-result v0
return v0
.end method
.method public getScale()F
.locals 1
.annotation runtime Landroid/view/ViewDebug$ExportedProperty;
category = "webview"
.end annotation
.annotation runtime Ljava/lang/Deprecated;
.end annotation
.prologue
.line 1037
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1038
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getScale()F
move-result v0
return v0
.end method
.method public getSettings()Landroid/webkit/WebSettings;
.locals 1
.prologue
.line 1559
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1560
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getSettings()Landroid/webkit/WebSettings;
move-result-object v0
return-object v0
.end method
.method public getTitle()Ljava/lang/String;
.locals 1
.annotation runtime Landroid/view/ViewDebug$ExportedProperty;
category = "webview"
.end annotation
.prologue
.line 1155
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1156
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getTitle()Ljava/lang/String;
move-result-object v0
return-object v0
.end method
.method public getTouchIconUrl()Ljava/lang/String;
.locals 1
.prologue
.line 1178
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getTouchIconUrl()Ljava/lang/String;
move-result-object v0
return-object v0
.end method
.method public getUrl()Ljava/lang/String;
.locals 1
.annotation runtime Landroid/view/ViewDebug$ExportedProperty;
category = "webview"
.end annotation
.prologue
.line 1128
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1129
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getUrl()Ljava/lang/String;
move-result-object v0
return-object v0
.end method
.method public getVisibleTitleHeight()I
.locals 1
.prologue
.line 561
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 562
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getVisibleTitleHeight()I
move-result v0
return v0
.end method
.method public getWebViewProvider()Landroid/webkit/WebViewProvider;
.locals 1
.prologue
.line 1747
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
return-object v0
.end method
.method public getZoomControls()Landroid/view/View;
.locals 1
.annotation runtime Ljava/lang/Deprecated;
.end annotation
.prologue
.line 1654
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1655
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getZoomControls()Landroid/view/View;
move-result-object v0
return-object v0
.end method
.method public goBack()V
.locals 1
.prologue
.line 920
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 921
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->goBack()V
.line 922
return-void
.end method
.method public goBackOrForward(I)V
.locals 1
.parameter "steps"
.prologue
.line 963
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 964
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider;->goBackOrForward(I)V
.line 965
return-void
.end method
.method public goForward()V
.locals 1
.prologue
.line 938
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 939
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->goForward()V
.line 940
return-void
.end method
.method public invokeZoomPicker()V
.locals 1
.prologue
.line 1062
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1063
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->invokeZoomPicker()V
.line 1064
return-void
.end method
.method public isPaused()Z
.locals 1
.prologue
.line 1259
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->isPaused()Z
move-result v0
return v0
.end method
.method public isPrivateBrowsingEnabled()Z
.locals 1
.prologue
.line 971
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 972
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->isPrivateBrowsingEnabled()Z
move-result v0
return v0
.end method
.method public loadData(Ljava/lang/String;Ljava/lang/String;Ljava/lang/String;)V
.locals 1
.parameter "data"
.parameter "mimeType"
.parameter "encoding"
.prologue
.line 830
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 831
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1, p2, p3}, Landroid/webkit/WebViewProvider;->loadData(Ljava/lang/String;Ljava/lang/String;Ljava/lang/String;)V
.line 832
return-void
.end method
.method public loadDataWithBaseURL(Ljava/lang/String;Ljava/lang/String;Ljava/lang/String;Ljava/lang/String;Ljava/lang/String;)V
.locals 6
.parameter "baseUrl"
.parameter "data"
.parameter "mimeType"
.parameter "encoding"
.parameter "historyUrl"
.prologue
.line 859
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 860
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
move-object v1, p1
move-object v2, p2
move-object v3, p3
move-object v4, p4
move-object v5, p5
invoke-interface/range {v0 .. v5}, Landroid/webkit/WebViewProvider;->loadDataWithBaseURL(Ljava/lang/String;Ljava/lang/String;Ljava/lang/String;Ljava/lang/String;Ljava/lang/String;)V
.line 861
return-void
.end method
.method public loadUrl(Ljava/lang/String;)V
.locals 1
.parameter "url"
.prologue
.line 783
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 784
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider;->loadUrl(Ljava/lang/String;)V
.line 785
return-void
.end method
.method public loadUrl(Ljava/lang/String;Ljava/util/Map;)V
.locals 1
.parameter "url"
.parameter
.annotation system Ldalvik/annotation/Signature;
value = {
"(",
"Ljava/lang/String;",
"Ljava/util/Map",
"<",
"Ljava/lang/String;",
"Ljava/lang/String;",
">;)V"
}
.end annotation
.prologue
.line 773
.local p2, additionalHttpHeaders:Ljava/util/Map;,"Ljava/util/Map<Ljava/lang/String;Ljava/lang/String;>;"
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 774
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1, p2}, Landroid/webkit/WebViewProvider;->loadUrl(Ljava/lang/String;Ljava/util/Map;)V
.line 775
return-void
.end method
.method protected onAttachedToWindow()V
.locals 1
.prologue
.line 1895
invoke-super {p0}, Landroid/widget/AbsoluteLayout;->onAttachedToWindow()V
.line 1896
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
invoke-interface {v0}, Landroid/webkit/WebViewProvider$ViewDelegate;->onAttachedToWindow()V
.line 1897
return-void
.end method
.method public onChildViewAdded(Landroid/view/View;Landroid/view/View;)V
.locals 0
.parameter "parent"
.parameter "child"
.annotation runtime Ljava/lang/Deprecated;
.end annotation
.prologue
.line 1604
return-void
.end method
.method public onChildViewRemoved(Landroid/view/View;Landroid/view/View;)V
.locals 0
.parameter "p"
.parameter "child"
.annotation runtime Ljava/lang/Deprecated;
.end annotation
.prologue
.line 1613
return-void
.end method
.method protected onConfigurationChanged(Landroid/content/res/Configuration;)V
.locals 1
.parameter "newConfig"
.prologue
.line 2064
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider$ViewDelegate;->onConfigurationChanged(Landroid/content/res/Configuration;)V
.line 2065
return-void
.end method
.method public onCreateInputConnection(Landroid/view/inputmethod/EditorInfo;)Landroid/view/inputmethod/InputConnection;
.locals 1
.parameter "outAttrs"
.prologue
.line 2069
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider$ViewDelegate;->onCreateInputConnection(Landroid/view/inputmethod/EditorInfo;)Landroid/view/inputmethod/InputConnection;
move-result-object v0
return-object v0
.end method
.method protected onDetachedFromWindow()V
.locals 1
.prologue
.line 1901
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
invoke-interface {v0}, Landroid/webkit/WebViewProvider$ViewDelegate;->onDetachedFromWindow()V
.line 1902
invoke-super {p0}, Landroid/widget/AbsoluteLayout;->onDetachedFromWindow()V
.line 1903
return-void
.end method
.method protected onDraw(Landroid/graphics/Canvas;)V
.locals 1
.parameter "canvas"
.prologue
.line 2054
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider$ViewDelegate;->onDraw(Landroid/graphics/Canvas;)V
.line 2055
return-void
.end method
.method protected onDrawVerticalScrollBar(Landroid/graphics/Canvas;Landroid/graphics/drawable/Drawable;IIII)V
.locals 7
.parameter "canvas"
.parameter "scrollBar"
.parameter "l"
.parameter "t"
.parameter "r"
.parameter "b"
.prologue
.line 2038
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
move-object v1, p1
move-object v2, p2
move v3, p3
move v4, p4
move v5, p5
move v6, p6
invoke-interface/range {v0 .. v6}, Landroid/webkit/WebViewProvider$ViewDelegate;->onDrawVerticalScrollBar(Landroid/graphics/Canvas;Landroid/graphics/drawable/Drawable;IIII)V
.line 2039
return-void
.end method
.method protected onFocusChanged(ZILandroid/graphics/Rect;)V
.locals 1
.parameter "focused"
.parameter "direction"
.parameter "previouslyFocusedRect"
.prologue
.line 2086
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
invoke-interface {v0, p1, p2, p3}, Landroid/webkit/WebViewProvider$ViewDelegate;->onFocusChanged(ZILandroid/graphics/Rect;)V
.line 2087
invoke-super {p0, p1, p2, p3}, Landroid/widget/AbsoluteLayout;->onFocusChanged(ZILandroid/graphics/Rect;)V
.line 2088
return-void
.end method
.method public onGenericMotionEvent(Landroid/view/MotionEvent;)Z
.locals 1
.parameter "event"
.prologue
.line 1968
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider$ViewDelegate;->onGenericMotionEvent(Landroid/view/MotionEvent;)Z
move-result v0
return v0
.end method
.method public onGlobalFocusChanged(Landroid/view/View;Landroid/view/View;)V
.locals 0
.parameter "oldFocus"
.parameter "newFocus"
.annotation runtime Ljava/lang/Deprecated;
.end annotation
.prologue
.line 1623
return-void
.end method
.method public onHoverEvent(Landroid/view/MotionEvent;)Z
.locals 1
.parameter "event"
.prologue
.line 1958
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider$ViewDelegate;->onHoverEvent(Landroid/view/MotionEvent;)Z
move-result v0
return v0
.end method
.method public onInitializeAccessibilityEvent(Landroid/view/accessibility/AccessibilityEvent;)V
.locals 1
.parameter "event"
.prologue
.line 2024
invoke-super {p0, p1}, Landroid/widget/AbsoluteLayout;->onInitializeAccessibilityEvent(Landroid/view/accessibility/AccessibilityEvent;)V
.line 2025
const-class v0, Landroid/webkit/WebView;
invoke-virtual {v0}, Ljava/lang/Class;->getName()Ljava/lang/String;
move-result-object v0
invoke-virtual {p1, v0}, Landroid/view/accessibility/AccessibilityEvent;->setClassName(Ljava/lang/CharSequence;)V
.line 2026
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider$ViewDelegate;->onInitializeAccessibilityEvent(Landroid/view/accessibility/AccessibilityEvent;)V
.line 2027
return-void
.end method
.method public onInitializeAccessibilityNodeInfo(Landroid/view/accessibility/AccessibilityNodeInfo;)V
.locals 1
.parameter "info"
.prologue
.line 2017
invoke-super {p0, p1}, Landroid/widget/AbsoluteLayout;->onInitializeAccessibilityNodeInfo(Landroid/view/accessibility/AccessibilityNodeInfo;)V
.line 2018
const-class v0, Landroid/webkit/WebView;
invoke-virtual {v0}, Ljava/lang/Class;->getName()Ljava/lang/String;
move-result-object v0
invoke-virtual {p1, v0}, Landroid/view/accessibility/AccessibilityNodeInfo;->setClassName(Ljava/lang/CharSequence;)V
.line 2019
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider$ViewDelegate;->onInitializeAccessibilityNodeInfo(Landroid/view/accessibility/AccessibilityNodeInfo;)V
.line 2020
return-void
.end method
.method public onKeyDown(ILandroid/view/KeyEvent;)Z
.locals 1
.parameter "keyCode"
.parameter "event"
.prologue
.line 1978
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
invoke-interface {v0, p1, p2}, Landroid/webkit/WebViewProvider$ViewDelegate;->onKeyDown(ILandroid/view/KeyEvent;)Z
move-result v0
return v0
.end method
.method public onKeyMultiple(IILandroid/view/KeyEvent;)Z
.locals 1
.parameter "keyCode"
.parameter "repeatCount"
.parameter "event"
.prologue
.line 1988
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
invoke-interface {v0, p1, p2, p3}, Landroid/webkit/WebViewProvider$ViewDelegate;->onKeyMultiple(IILandroid/view/KeyEvent;)Z
move-result v0
return v0
.end method
.method public onKeyUp(ILandroid/view/KeyEvent;)Z
.locals 1
.parameter "keyCode"
.parameter "event"
.prologue
.line 1983
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
invoke-interface {v0, p1, p2}, Landroid/webkit/WebViewProvider$ViewDelegate;->onKeyUp(ILandroid/view/KeyEvent;)Z
move-result v0
return v0
.end method
.method protected onMeasure(II)V
.locals 1
.parameter "widthMeasureSpec"
.parameter "heightMeasureSpec"
.annotation runtime Ljava/lang/Deprecated;
.end annotation
.prologue
.line 2121
invoke-super {p0, p1, p2}, Landroid/widget/AbsoluteLayout;->onMeasure(II)V
.line 2122
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
invoke-interface {v0, p1, p2}, Landroid/webkit/WebViewProvider$ViewDelegate;->onMeasure(II)V
.line 2123
return-void
.end method
.method protected onOverScrolled(IIZZ)V
.locals 1
.parameter "scrollX"
.parameter "scrollY"
.parameter "clampedX"
.parameter "clampedY"
.prologue
.line 2043
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
invoke-interface {v0, p1, p2, p3, p4}, Landroid/webkit/WebViewProvider$ViewDelegate;->onOverScrolled(IIZZ)V
.line 2044
return-void
.end method
.method public onPause()V
.locals 1
.prologue
.line 1240
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1241
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->onPause()V
.line 1242
return-void
.end method
.method public onResume()V
.locals 1
.prologue
.line 1248
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1249
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->onResume()V
.line 1250
return-void
.end method
.method protected onScrollChanged(IIII)V
.locals 1
.parameter "l"
.parameter "t"
.parameter "oldl"
.parameter "oldt"
.prologue
.line 2104
invoke-super {p0, p1, p2, p3, p4}, Landroid/widget/AbsoluteLayout;->onScrollChanged(IIII)V
.line 2105
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
invoke-interface {v0, p1, p2, p3, p4}, Landroid/webkit/WebViewProvider$ViewDelegate;->onScrollChanged(IIII)V
.line 2106
return-void
.end method
.method protected onSizeChanged(IIII)V
.locals 1
.parameter "w"
.parameter "h"
.parameter "ow"
.parameter "oh"
.prologue
.line 2098
invoke-super {p0, p1, p2, p3, p4}, Landroid/widget/AbsoluteLayout;->onSizeChanged(IIII)V
.line 2099
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
invoke-interface {v0, p1, p2, p3, p4}, Landroid/webkit/WebViewProvider$ViewDelegate;->onSizeChanged(IIII)V
.line 2100
return-void
.end method
.method public onTouchEvent(Landroid/view/MotionEvent;)Z
.locals 1
.parameter "event"
.prologue
.line 1963
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider$ViewDelegate;->onTouchEvent(Landroid/view/MotionEvent;)Z
move-result v0
return v0
.end method
.method public onTrackballEvent(Landroid/view/MotionEvent;)Z
.locals 1
.parameter "event"
.prologue
.line 1973
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider$ViewDelegate;->onTrackballEvent(Landroid/view/MotionEvent;)Z
move-result v0
return v0
.end method
.method protected onVisibilityChanged(Landroid/view/View;I)V
.locals 1
.parameter "changedView"
.parameter "visibility"
.prologue
.line 2074
invoke-super {p0, p1, p2}, Landroid/widget/AbsoluteLayout;->onVisibilityChanged(Landroid/view/View;I)V
.line 2075
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
invoke-interface {v0, p1, p2}, Landroid/webkit/WebViewProvider$ViewDelegate;->onVisibilityChanged(Landroid/view/View;I)V
.line 2076
return-void
.end method
.method public onWindowFocusChanged(Z)V
.locals 1
.parameter "hasWindowFocus"
.prologue
.line 2080
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider$ViewDelegate;->onWindowFocusChanged(Z)V
.line 2081
invoke-super {p0, p1}, Landroid/widget/AbsoluteLayout;->onWindowFocusChanged(Z)V
.line 2082
return-void
.end method
.method protected onWindowVisibilityChanged(I)V
.locals 1
.parameter "visibility"
.prologue
.line 2048
invoke-super {p0, p1}, Landroid/widget/AbsoluteLayout;->onWindowVisibilityChanged(I)V
.line 2049
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider$ViewDelegate;->onWindowVisibilityChanged(I)V
.line 2050
return-void
.end method
.method public overlayHorizontalScrollbar()Z
.locals 1
.prologue
.line 540
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 541
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->overlayHorizontalScrollbar()Z
move-result v0
return v0
.end method
.method public overlayVerticalScrollbar()Z
.locals 1
.prologue
.line 550
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 551
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->overlayVerticalScrollbar()Z
move-result v0
return v0
.end method
.method public pageDown(Z)Z
.locals 1
.parameter "bottom"
.prologue
.line 993
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 994
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider;->pageDown(Z)Z
move-result v0
return v0
.end method
.method public pageUp(Z)Z
.locals 1
.parameter "top"
.prologue
.line 982
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 983
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider;->pageUp(Z)Z
move-result v0
return v0
.end method
.method public pauseTimers()V
.locals 1
.prologue
.line 1219
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1220
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->pauseTimers()V
.line 1221
return-void
.end method
.method public performAccessibilityAction(ILandroid/os/Bundle;)Z
.locals 1
.parameter "action"
.parameter "arguments"
.prologue
.line 2031
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
invoke-interface {v0, p1, p2}, Landroid/webkit/WebViewProvider$ViewDelegate;->performAccessibilityAction(ILandroid/os/Bundle;)Z
move-result v0
return v0
.end method
.method public performLongClick()Z
.locals 1
.prologue
.line 2059
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
invoke-interface {v0}, Landroid/webkit/WebViewProvider$ViewDelegate;->performLongClick()Z
move-result v0
return v0
.end method
.method public postUrl(Ljava/lang/String;[B)V
.locals 1
.parameter "url"
.parameter "postData"
.prologue
.line 796
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 797
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1, p2}, Landroid/webkit/WebViewProvider;->postUrl(Ljava/lang/String;[B)V
.line 798
return-void
.end method
.method public refreshPlugins(Z)V
.locals 0
.parameter "reloadOpenPages"
.annotation runtime Ljava/lang/Deprecated;
.end annotation
.prologue
.line 1582
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1583
return-void
.end method
.method public reload()V
.locals 1
.prologue
.line 902
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 903
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->reload()V
.line 904
return-void
.end method
.method public removeJavascriptInterface(Ljava/lang/String;)V
.locals 1
.parameter "name"
.prologue
.line 1547
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1548
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider;->removeJavascriptInterface(Ljava/lang/String;)V
.line 1549
return-void
.end method
.method public requestChildRectangleOnScreen(Landroid/view/View;Landroid/graphics/Rect;Z)Z
.locals 1
.parameter "child"
.parameter "rect"
.parameter "immediate"
.prologue
.line 2127
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
invoke-interface {v0, p1, p2, p3}, Landroid/webkit/WebViewProvider$ViewDelegate;->requestChildRectangleOnScreen(Landroid/view/View;Landroid/graphics/Rect;Z)Z
move-result v0
return v0
.end method
.method public requestFocus(ILandroid/graphics/Rect;)Z
.locals 1
.parameter "direction"
.parameter "previouslyFocusedRect"
.prologue
.line 2115
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
invoke-interface {v0, p1, p2}, Landroid/webkit/WebViewProvider$ViewDelegate;->requestFocus(ILandroid/graphics/Rect;)Z
move-result v0
return v0
.end method
.method public requestFocusNodeHref(Landroid/os/Message;)V
.locals 1
.parameter "hrefMsg"
.prologue
.line 1103
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1104
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider;->requestFocusNodeHref(Landroid/os/Message;)V
.line 1105
return-void
.end method
.method public requestImageRef(Landroid/os/Message;)V
.locals 1
.parameter "msg"
.prologue
.line 1115
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1116
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider;->requestImageRef(Landroid/os/Message;)V
.line 1117
return-void
.end method
.method public restorePicture(Landroid/os/Bundle;Ljava/io/File;)Z
.locals 1
.parameter "b"
.parameter "src"
.annotation runtime Ljava/lang/Deprecated;
.end annotation
.prologue
.line 740
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 741
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1, p2}, Landroid/webkit/WebViewProvider;->restorePicture(Landroid/os/Bundle;Ljava/io/File;)Z
move-result v0
return v0
.end method
.method public restoreState(Landroid/os/Bundle;)Landroid/webkit/WebBackForwardList;
.locals 1
.parameter "inState"
.prologue
.line 757
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 758
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider;->restoreState(Landroid/os/Bundle;)Landroid/webkit/WebBackForwardList;
move-result-object v0
return-object v0
.end method
.method public resumeTimers()V
.locals 1
.prologue
.line 1228
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1229
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->resumeTimers()V
.line 1230
return-void
.end method
.method public savePassword(Ljava/lang/String;Ljava/lang/String;Ljava/lang/String;)V
.locals 1
.parameter "host"
.parameter "username"
.parameter "password"
.prologue
.line 605
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 606
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1, p2, p3}, Landroid/webkit/WebViewProvider;->savePassword(Ljava/lang/String;Ljava/lang/String;Ljava/lang/String;)V
.line 607
return-void
.end method
.method public savePicture(Landroid/os/Bundle;Ljava/io/File;)Z
.locals 1
.parameter "b"
.parameter "dest"
.annotation runtime Ljava/lang/Deprecated;
.end annotation
.prologue
.line 723
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 724
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1, p2}, Landroid/webkit/WebViewProvider;->savePicture(Landroid/os/Bundle;Ljava/io/File;)Z
move-result v0
return v0
.end method
.method public saveState(Landroid/os/Bundle;)Landroid/webkit/WebBackForwardList;
.locals 1
.parameter "outState"
.prologue
.line 707
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 708
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider;->saveState(Landroid/os/Bundle;)Landroid/webkit/WebBackForwardList;
move-result-object v0
return-object v0
.end method
.method public saveWebArchive(Ljava/lang/String;)V
.locals 1
.parameter "filename"
.prologue
.line 869
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 870
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider;->saveWebArchive(Ljava/lang/String;)V
.line 871
return-void
.end method
.method public saveWebArchive(Ljava/lang/String;ZLandroid/webkit/ValueCallback;)V
.locals 1
.parameter "basename"
.parameter "autoname"
.parameter
.annotation system Ldalvik/annotation/Signature;
value = {
"(",
"Ljava/lang/String;",
"Z",
"Landroid/webkit/ValueCallback",
"<",
"Ljava/lang/String;",
">;)V"
}
.end annotation
.prologue
.line 886
.local p3, callback:Landroid/webkit/ValueCallback;,"Landroid/webkit/ValueCallback<Ljava/lang/String;>;"
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 887
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1, p2, p3}, Landroid/webkit/WebViewProvider;->saveWebArchive(Ljava/lang/String;ZLandroid/webkit/ValueCallback;)V
.line 888
return-void
.end method
.method public setBackgroundColor(I)V
.locals 1
.parameter "color"
.prologue
.line 2132
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider$ViewDelegate;->setBackgroundColor(I)V
.line 2133
return-void
.end method
.method public setCertificate(Landroid/net/http/SslCertificate;)V
.locals 1
.parameter "certificate"
.annotation runtime Ljava/lang/Deprecated;
.end annotation
.prologue
.line 584
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 585
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider;->setCertificate(Landroid/net/http/SslCertificate;)V
.line 586
return-void
.end method
.method public setDownloadListener(Landroid/webkit/DownloadListener;)V
.locals 1
.parameter "listener"
.prologue
.line 1461
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1462
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider;->setDownloadListener(Landroid/webkit/DownloadListener;)V
.line 1463
return-void
.end method
.method public setFindListener(Landroid/webkit/WebView$FindListener;)V
.locals 1
.parameter "listener"
.prologue
.line 1331
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1332
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider;->setFindListener(Landroid/webkit/WebView$FindListener;)V
.line 1333
return-void
.end method
.method protected setFrame(IIII)Z
.locals 1
.parameter "left"
.parameter "top"
.parameter "right"
.parameter "bottom"
.prologue
.line 2093
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
invoke-interface {v0, p1, p2, p3, p4}, Landroid/webkit/WebViewProvider$ViewDelegate;->setFrame(IIII)Z
move-result v0
return v0
.end method
.method public setHorizontalScrollbarOverlay(Z)V
.locals 1
.parameter "overlay"
.prologue
.line 520
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 521
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider;->setHorizontalScrollbarOverlay(Z)V
.line 522
return-void
.end method
.method public setHttpAuthUsernamePassword(Ljava/lang/String;Ljava/lang/String;Ljava/lang/String;Ljava/lang/String;)V
.locals 1
.parameter "host"
.parameter "realm"
.parameter "username"
.parameter "password"
.prologue
.line 624
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 625
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1, p2, p3, p4}, Landroid/webkit/WebViewProvider;->setHttpAuthUsernamePassword(Ljava/lang/String;Ljava/lang/String;Ljava/lang/String;Ljava/lang/String;)V
.line 626
return-void
.end method
.method public setInitialScale(I)V
.locals 1
.parameter "scaleInPercent"
.prologue
.line 1052
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1053
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider;->setInitialScale(I)V
.line 1054
return-void
.end method
.method public setLayerType(ILandroid/graphics/Paint;)V
.locals 1
.parameter "layerType"
.parameter "paint"
.prologue
.line 2137
invoke-super {p0, p1, p2}, Landroid/widget/AbsoluteLayout;->setLayerType(ILandroid/graphics/Paint;)V
.line 2138
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
invoke-interface {v0, p1, p2}, Landroid/webkit/WebViewProvider$ViewDelegate;->setLayerType(ILandroid/graphics/Paint;)V
.line 2139
return-void
.end method
.method public setLayoutParams(Landroid/view/ViewGroup$LayoutParams;)V
.locals 1
.parameter "params"
.prologue
.line 1907
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider$ViewDelegate;->setLayoutParams(Landroid/view/ViewGroup$LayoutParams;)V
.line 1908
return-void
.end method
.method public setMapTrackballToArrowKeys(Z)V
.locals 1
.parameter "setMap"
.annotation runtime Ljava/lang/Deprecated;
.end annotation
.prologue
.line 1630
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1631
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider;->setMapTrackballToArrowKeys(Z)V
.line 1632
return-void
.end method
.method public setNetworkAvailable(Z)V
.locals 1
.parameter "networkUp"
.prologue
.line 691
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 692
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider;->setNetworkAvailable(Z)V
.line 693
return-void
.end method
.method public setOverScrollMode(I)V
.locals 1
.parameter "mode"
.prologue
.line 1912
invoke-super {p0, p1}, Landroid/widget/AbsoluteLayout;->setOverScrollMode(I)V
.line 1916
invoke-direct {p0}, Landroid/webkit/WebView;->ensureProviderCreated()V
.line 1917
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider$ViewDelegate;->setOverScrollMode(I)V
.line 1918
return-void
.end method
.method public setPictureListener(Landroid/webkit/WebView$PictureListener;)V
.locals 1
.parameter "listener"
.annotation runtime Ljava/lang/Deprecated;
.end annotation
.prologue
.line 1486
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1487
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider;->setPictureListener(Landroid/webkit/WebView$PictureListener;)V
.line 1488
return-void
.end method
.method public setScrollBarStyle(I)V
.locals 1
.parameter "style"
.prologue
.line 1922
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider$ViewDelegate;->setScrollBarStyle(I)V
.line 1923
invoke-super {p0, p1}, Landroid/widget/AbsoluteLayout;->setScrollBarStyle(I)V
.line 1924
return-void
.end method
.method public setVerticalScrollbarOverlay(Z)V
.locals 1
.parameter "overlay"
.prologue
.line 530
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 531
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider;->setVerticalScrollbarOverlay(Z)V
.line 532
return-void
.end method
.method public setWebChromeClient(Landroid/webkit/WebChromeClient;)V
.locals 1
.parameter "client"
.prologue
.line 1473
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1474
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider;->setWebChromeClient(Landroid/webkit/WebChromeClient;)V
.line 1475
return-void
.end method
.method public setWebViewClient(Landroid/webkit/WebViewClient;)V
.locals 1
.parameter "client"
.prologue
.line 1449
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1450
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1}, Landroid/webkit/WebViewProvider;->setWebViewClient(Landroid/webkit/WebViewClient;)V
.line 1451
return-void
.end method
.method public shouldDelayChildPressedState()Z
.locals 1
.annotation runtime Ljava/lang/Deprecated;
.end annotation
.prologue
.line 2012
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->getViewDelegate()Landroid/webkit/WebViewProvider$ViewDelegate;
move-result-object v0
invoke-interface {v0}, Landroid/webkit/WebViewProvider$ViewDelegate;->shouldDelayChildPressedState()Z
move-result v0
return v0
.end method
.method public showFindDialog(Ljava/lang/String;Z)Z
.locals 1
.parameter "text"
.parameter "showIme"
.prologue
.line 1391
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1392
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0, p1, p2}, Landroid/webkit/WebViewProvider;->showFindDialog(Ljava/lang/String;Z)Z
move-result v0
return v0
.end method
.method public stopLoading()V
.locals 1
.prologue
.line 894
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 895
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->stopLoading()V
.line 896
return-void
.end method
.method public zoomIn()Z
.locals 1
.prologue
.line 1694
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1695
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->zoomIn()Z
move-result v0
return v0
.end method
.method public zoomOut()Z
.locals 1
.prologue
.line 1704
invoke-static {}, Landroid/webkit/WebView;->checkThread()V
.line 1705
iget-object v0, p0, Landroid/webkit/WebView;->mProvider:Landroid/webkit/WebViewProvider;
invoke-interface {v0}, Landroid/webkit/WebViewProvider;->zoomOut()Z
move-result v0
return v0
.end method
| {
"content_hash": "f921ee7240f1f2ca1665127c331b2ecf",
"timestamp": "",
"source": "github",
"line_count": 3149,
"max_line_length": 185,
"avg_line_length": 25.984757065735153,
"alnum_prop": 0.7174981057365629,
"repo_name": "baidurom/reference",
"id": "4fbdb1cd9b086bfb2dc217d18f028ec1dfc739ab",
"size": "81826",
"binary": false,
"copies": "1",
"ref": "refs/heads/coron-4.2",
"path": "bosp/framework.jar.out/smali/android/webkit/WebView.smali",
"mode": "33188",
"license": "apache-2.0",
"language": [],
"symlink_target": ""
} |
package org.springframework.cloud.task.listener;
import org.junit.Test;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.context.PropertyPlaceholderAutoConfiguration;
import org.springframework.boot.autoconfigure.jdbc.EmbeddedDataSourceConfiguration;
import org.springframework.cloud.stream.test.binder.TestSupportBinderAutoConfiguration;
import org.springframework.cloud.task.configuration.EnableTask;
import org.springframework.context.ConfigurableApplicationContext;
import org.springframework.context.annotation.Configuration;
import static org.junit.Assert.assertNotNull;
/**
* @author Michael Minella
* @author Ilayaperumal Gopinathan
* @author Glenn Renfro
*/
public class TaskEventTests {
private static final String TASK_NAME = "taskEventTest";
@Test
public void testDefaultConfiguration() {
ConfigurableApplicationContext applicationContext =
SpringApplication.run(new Class[]{PropertyPlaceholderAutoConfiguration.class,EmbeddedDataSourceConfiguration.class,TaskEventsConfiguration.class,
TaskEventAutoConfiguration.class,
TestSupportBinderAutoConfiguration.class},
new String[]{ "--spring.cloud.task.closecontext_enable=false",
"--spring.main.web-environment=false"});
assertNotNull(applicationContext.getBean("taskEventListener"));
assertNotNull(applicationContext.getBean(TaskEventAutoConfiguration.TaskEventChannels.class));
}
@Configuration
@EnableTask
public static class TaskEventsConfiguration {
}
}
| {
"content_hash": "138707fc64c472b159dfaa0168eb9179",
"timestamp": "",
"source": "github",
"line_count": 43,
"max_line_length": 149,
"avg_line_length": 35.53488372093023,
"alnum_prop": 0.8232984293193717,
"repo_name": "trisberg/spring-cloud-task",
"id": "318e41a697ba32e02d3ab1f6d24eed3e37ed61a5",
"size": "2143",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "spring-cloud-task-stream/src/test/java/org/springframework/cloud/task/listener/TaskEventTests.java",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "Batchfile",
"bytes": "40228"
},
{
"name": "CSS",
"bytes": "5654"
},
{
"name": "Java",
"bytes": "655150"
},
{
"name": "Ruby",
"bytes": "423"
},
{
"name": "Shell",
"bytes": "57060"
},
{
"name": "XSLT",
"bytes": "33701"
}
],
"symlink_target": ""
} |
module GoodData
# Project Not Found
class ValidationError < RuntimeError
DEFAULT_MSG = 'Validation has failed. Run validate method on blueprint to learn more about the errors.'
def initialize(msg = DEFAULT_MSG)
super(msg)
end
end
end
| {
"content_hash": "5388ab5aca57dc931392629d170206ac",
"timestamp": "",
"source": "github",
"line_count": 10,
"max_line_length": 107,
"avg_line_length": 25.9,
"alnum_prop": 0.7181467181467182,
"repo_name": "korczis/app_store",
"id": "0289fbc1b7480b6eb74f3841a5d08dbb2df6151e",
"size": "278",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "apps/user_filters_brick/vendor/gooddata-0.6.20/lib/gooddata/exceptions/validation_error.rb",
"mode": "33188",
"license": "bsd-3-clause",
"language": [
{
"name": "CSS",
"bytes": "5089"
},
{
"name": "HTML",
"bytes": "7931"
},
{
"name": "JavaScript",
"bytes": "1326276"
},
{
"name": "Ruby",
"bytes": "78091"
}
],
"symlink_target": ""
} |
// Copyright (c) 2011-2017 The Machinecoin Core developers
// Distributed under the MIT software license, see the accompanying
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
#ifndef MACHINECOIN_QT_SENDCOINSDIALOG_H
#define MACHINECOIN_QT_SENDCOINSDIALOG_H
#include <qt/walletmodel.h>
#include <QDialog>
#include <QMessageBox>
#include <QString>
#include <QTimer>
class ClientModel;
class PlatformStyle;
class SendCoinsEntry;
class SendCoinsRecipient;
namespace Ui {
class SendCoinsDialog;
}
QT_BEGIN_NAMESPACE
class QUrl;
QT_END_NAMESPACE
/** Dialog for sending machinecoins */
class SendCoinsDialog : public QDialog
{
Q_OBJECT
public:
explicit SendCoinsDialog(const PlatformStyle *platformStyle, QWidget *parent = 0);
~SendCoinsDialog();
void setClientModel(ClientModel *clientModel);
void setModel(WalletModel *model);
/** Set up the tab chain manually, as Qt messes up the tab chain by default in some cases (issue https://bugreports.qt-project.org/browse/QTBUG-10907).
*/
QWidget *setupTabChain(QWidget *prev);
void setAddress(const QString &address);
void pasteEntry(const SendCoinsRecipient &rv);
bool handlePaymentRequest(const SendCoinsRecipient &recipient);
public Q_SLOTS:
void clear();
void reject();
void accept();
SendCoinsEntry *addEntry();
void updateTabsAndLabels();
void setBalance(const interfaces::WalletBalances& balances);
Q_SIGNALS:
void coinsSent(const uint256& txid);
private:
Ui::SendCoinsDialog *ui;
ClientModel *clientModel;
WalletModel *model;
bool fNewRecipientAllowed;
bool fFeeMinimized;
const PlatformStyle *platformStyle;
// Process WalletModel::SendCoinsReturn and generate a pair consisting
// of a message and message flags for use in Q_EMIT message().
// Additional parameter msgArg can be used via .arg(msgArg).
void processSendCoinsReturn(const WalletModel::SendCoinsReturn &sendCoinsReturn, const QString &msgArg = QString());
void minimizeFeeSection(bool fMinimize);
void updateFeeMinimizedLabel();
// Update the passed in CCoinControl with state from the GUI
void updateCoinControlState(CCoinControl& ctrl);
private Q_SLOTS:
void on_sendButton_clicked();
void on_buttonChooseFee_clicked();
void on_buttonMinimizeFee_clicked();
void removeEntry(SendCoinsEntry* entry);
void useAvailableBalance(SendCoinsEntry* entry);
void updateDisplayUnit();
void coinControlFeatureChanged(bool);
void coinControlButtonClicked();
void coinControlChangeChecked(int);
void coinControlChangeEdited(const QString &);
void coinControlUpdateLabels();
void coinControlClipboardQuantity();
void coinControlClipboardAmount();
void coinControlClipboardFee();
void coinControlClipboardAfterFee();
void coinControlClipboardBytes();
void coinControlClipboardLowOutput();
void coinControlClipboardChange();
void setMinimumFee();
void updateFeeSectionControls();
void updateMinFeeLabel();
void updateSmartFeeLabel();
Q_SIGNALS:
// Fired when a message should be reported to the user
void message(const QString &title, const QString &message, unsigned int style);
};
#define SEND_CONFIRM_DELAY 3
class SendConfirmationDialog : public QMessageBox
{
Q_OBJECT
public:
SendConfirmationDialog(const QString &title, const QString &text, int secDelay = SEND_CONFIRM_DELAY, QWidget *parent = 0);
int exec();
private Q_SLOTS:
void countDown();
void updateYesButton();
private:
QAbstractButton *yesButton;
QTimer countDownTimer;
int secDelay;
};
#endif // MACHINECOIN_QT_SENDCOINSDIALOG_H
| {
"content_hash": "eb54dac0040f30cbc7f47a843c94c137",
"timestamp": "",
"source": "github",
"line_count": 126,
"max_line_length": 155,
"avg_line_length": 29.365079365079364,
"alnum_prop": 0.7424324324324324,
"repo_name": "machinecoin-project/machinecoin",
"id": "6766cbb92f8d114f3c65c09ab0945b10b9f940e2",
"size": "3700",
"binary": false,
"copies": "2",
"ref": "refs/heads/0.17",
"path": "src/qt/sendcoinsdialog.h",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "Assembly",
"bytes": "7639"
},
{
"name": "C",
"bytes": "342684"
},
{
"name": "C++",
"bytes": "3521961"
},
{
"name": "CSS",
"bytes": "1127"
},
{
"name": "Groff",
"bytes": "18048"
},
{
"name": "HTML",
"bytes": "50621"
},
{
"name": "Java",
"bytes": "2100"
},
{
"name": "Makefile",
"bytes": "66797"
},
{
"name": "Objective-C",
"bytes": "2023"
},
{
"name": "Objective-C++",
"bytes": "7246"
},
{
"name": "Protocol Buffer",
"bytes": "2308"
},
{
"name": "Python",
"bytes": "211880"
},
{
"name": "QMake",
"bytes": "2019"
},
{
"name": "Shell",
"bytes": "40513"
}
],
"symlink_target": ""
} |
/* line 1, static/base.scss */
body {
background-attachment: fixed;
background-size: cover;
font-size: 16px;
font-weight: 300;
font-family: 'Open Sans', sans-serif;
padding-bottom: 30px; }
/* line 9, static/base.scss */
body h1, body h2, body h3, body h4, body h5, body h6 {
font-family: 'Muli', sans-serif;
font-weight: 200;
font-style: italic; }
/* line 15, static/base.scss */
body > .container {
width: auto;
max-width: 940px; }
/* line 19, static/base.scss */
body > .container h1 small {
color: #b8bcc0; }
/* line 24, static/base.scss */
body header {
position: fixed;
top: 0;
height: 50px;
width: 100%;
z-index: 10001; }
/* line 31, static/base.scss */
body header nav {
font-family: "Muli", sans-serif;
text-align: center;
box-shadow: 0 0 4px rgba(0, 0, 0, 0.07), 0 4px 8px rgba(0, 0, 0, 0.14); }
/* line 36, static/base.scss */
body header nav .navbar-header {
width: 200px; }
/* line 39, static/base.scss */
body header nav .navbar-header .navbar-brand {
font-style: italic;
font-weight: 300;
color: #337ab7; }
/* line 44, static/base.scss */
body header nav .navbar-header .navbar-brand:focus, body header nav .navbar-header .navbar-brand:hover {
color: #286090; }
/* line 51, static/base.scss */
body header nav > .container-fluid {
list-style: none;
margin: 0;
overflow: scroll;
padding: 0;
top: 0; }
/* line 59, static/base.scss */
body header nav #NavTrigger {
height: 50px;
padding-top: 16px;
position: absolute;
right: 0;
text-align: left;
top: 0;
width: 40px; }
/* line 69, static/base.scss */
body header nav #NavTrigger:before,
body header nav #NavTrigger:after,
body header nav #NavTrigger span {
background: #4c4c4c;
border-radius: 50%;
content: '.';
height: 4px;
line-height: 4px;
margin: 2px auto;
width: 4px;
display: block;
overflow: hidden;
text-indent: -9999px; }
/* line 86, static/base.scss */
body #ShadowBox {
background: rgba(51, 51, 51, 0.8);
content: '[]';
display: none;
height: 100vh;
left: 0;
position: absolute;
text-indent: -9999px;
top: 0;
width: 100vw;
z-index: 98; }
/* line 99, static/base.scss */
body main {
color: #f8f8f8;
background-color: rgba(51, 51, 51, 0.9);
padding: 15px;
margin: 110px 0 30px 0; }
/* line 105, static/base.scss */
body main a {
color: #c9c3e7; }
/* line 108, static/base.scss */
body main a:hover, body main a:focus {
color: white; }
/* line 114, static/base.scss */
body main .alert {
box-shadow: 0 1px 1px rgba(0, 0, 0, 0.05);
margin-top: 0 !important; }
/* line 118, static/base.scss */
body main .alert.alert-default {
color: #252525;
background-color: #fff;
border-color: #ddd; }
/* line 124, static/base.scss */
body main .alert a {
color: #000;
text-decoration: underline; }
/* line 128, static/base.scss */
body main .alert a:hover, body main .alert a:focus {
text-decoration: none; }
/* line 135, static/base.scss */
body main .page-header {
margin-top: 0px; }
/* line 138, static/base.scss */
body main .page-header h1 {
margin-top: 10px; }
/* line 144, static/base.scss */
body .panel,
body .alert {
color: #252525; }
/* line 148, static/base.scss */
body .panel.panel-float, body .panel.alert-float,
body .alert.panel-float,
body .alert.alert-float {
max-width: 33%; }
/* line 152, static/base.scss */
body .panel.panel-float.panel-float-right, body .panel.panel-float.alert-float-right, body .panel.alert-float.panel-float-right, body .panel.alert-float.alert-float-right,
body .alert.panel-float.panel-float-right,
body .alert.panel-float.alert-float-right,
body .alert.alert-float.panel-float-right,
body .alert.alert-float.alert-float-right {
float: right;
margin: 1em 0 1em 1em; }
/* line 158, static/base.scss */
body .panel.panel-float.panel-float-left, body .panel.panel-float.alert-float-left, body .panel.alert-float.panel-float-left, body .panel.alert-float.alert-float-left,
body .alert.panel-float.panel-float-left,
body .alert.panel-float.alert-float-left,
body .alert.alert-float.panel-float-left,
body .alert.alert-float.alert-float-left {
float: left;
margin: 1em 1em 1em 0; }
/* line 165, static/base.scss */
body .panel a,
body .alert a {
color: #00009d; }
/* line 168, static/base.scss */
body .panel a.btn,
body .alert a.btn {
margin-top: 1em;
color: #00009d !important;
text-decoration: none; }
/* line 176, static/base.scss */
body .pull-glyph-right .glyphicon {
float: right;
margin-top: 2px;
margin-right: -6px;
margin-left: 6px; }
/* line 184, static/base.scss */
body.home {
background-image: url('/static/images/home.jpg');
background-color: #58c8f6; }
/* line 188, static/base.scss */
body.home main {
background-color: inherit; }
/* line 192, static/base.scss */
body.home > .container {
max-width: inherit; }
/* line 196, static/base.scss */
body.home .page-header {
margin-top: 40px; }
/* line 200, static/base.scss */
body.home div#sidebar {
background-color: rgba(51, 51, 51, 0.9);
color: #f8f8f8;
padding-bottom: 10px;
margin-top: 20px;
min-width: 250px; }
/* line 207, static/base.scss */
body.home div#sidebar .page-header {
margin-top: 20px; }
/* line 211, static/base.scss */
body.home div#sidebar .control-label {
display: none; }
/* line 217, static/base.scss */
body.story {
background-image: url('/static/images/story.jpg');
background-color: #87c1e9; }
/* line 222, static/base.scss */
body.detail {
background-image: url('/static/images/details.jpg');
background-color: #063d90; }
/* line 227, static/base.scss */
body.detail main .panel-body > .datetime,
body.detail main .panel-body > .address {
float: left;
display: block; }
/* line 235, static/base.scss */
body.gift {
background-image: url('/static/images/gifts.jpg');
background-color: #58c8f6; }
/* line 240, static/base.scss */
body.photo {
background-image: url('/static/images/photos.jpg');
background-color: #d8c5b7; }
/* line 245, static/base.scss */
body.colophon {
background-image: url('/static/images/colophon.jpg');
background-color: #063d90; }
/* line 250, static/base.scss */
body.guest {
background-image: url('/static/images/guest.jpg');
background-color: #0544ab; }
/* line 254, static/base.scss */
body.guest .intro {
padding-bottom: 20px; }
/* line 257, static/base.scss */
body.guest .intro p {
display: block;
font-size: large; }
/* line 264, static/base.scss */
body.guest #events dd + dt {
margin-top: 1em; }
/* line 277, static/base.scss */
body.home li.home a,
body.home li.home a:hover,
body.home li.home a:focus,
body.story li.story a,
body.story li.story a:hover,
body.story li.story a:focus,
body.detail li.detail a,
body.detail li.detail a:hover,
body.detail li.detail a:focus,
body.registry li.registry a,
body.registry li.registry a:hover,
body.registry li.registry a:focus,
body.photo li.photo a,
body.photo li.photo a:hover,
body.photo li.photo a:focus,
body.guest li.guest a,
body.guest li.guest a:hover,
body.guest li.guest a:focus,
body.colophon li.colophon a,
body.colophon li.colophon a:hover,
body.colophon li.colophon a:focus {
color: #555;
background-color: #e7e7e7; }
@media (min-width: 768px) {
/* line 286, static/base.scss */
#NavTrigger {
display: none; }
/* line 290, static/base.scss */
.navbar-header {
margin-right: -200px; }
/* line 294, static/base.scss */
body nav > .container-fluid {
top: 0;
overflow: hidden;
position: inherit;
display: inline-block;
transform: translate(0, 0);
width: auto; } }
@media (max-width: 1080px) and (min-width: 768px) {
/* line 305, static/base.scss */
.navbar-header {
display: none; } }
@media (max-width: 768px) {
/* line 313, static/base.scss */
body header nav .navbar-header {
position: fixed; }
/* line 317, static/base.scss */
body header nav > .container-fluid {
transform: translate(0, 0);
background-color: #f8f8f8;
right: 0;
display: none; }
/* line 326, static/base.scss */
body main h2 small {
display: none; }
/* line 332, static/base.scss */
body main .panel.panel-float, body main .panel.alert-float,
body main .alert.panel-float,
body main .alert.alert-float {
max-width: 100%;
width: 100%; }
/* line 337, static/base.scss */
body main .panel.panel-float.panel-float-right, body main .panel.panel-float.alert-float-right, body main .panel.alert-float.panel-float-right, body main .panel.alert-float.alert-float-right,
body main .alert.panel-float.panel-float-right,
body main .alert.panel-float.alert-float-right,
body main .alert.alert-float.panel-float-right,
body main .alert.alert-float.alert-float-right {
float: none;
margin: 1em 0 1em 0 !important; }
/* line 343, static/base.scss */
body main .panel.panel-float.panel-float-left, body main .panel.panel-float.alert-float-left, body main .panel.alert-float.panel-float-left, body main .panel.alert-float.alert-float-left,
body main .alert.panel-float.panel-float-left,
body main .alert.panel-float.alert-float-left,
body main .alert.alert-float.panel-float-left,
body main .alert.alert-float.alert-float-left {
float: none;
margin: 1em 0 1em 0 !important; }
/* line 352, static/base.scss */
body.home {
background-position-x: 45%; }
/* line 355, static/base.scss */
body.home #sidebar {
position: absolute;
width: 100%;
bottom: 0;
left: 0;
right: 0; }
/* line 365, static/base.scss */
body.home.nav-active #sidebar {
display: none; }
/* line 371, static/base.scss */
body.guest main .row > * {
min-width: 100%; }
/* line 379, static/base.scss */
body.detail main .panel-body > .datetime,
body.detail main .panel-body > .address {
float: left;
display: block;
max-width: 40%; }
/* line 386, static/base.scss */
body.detail main .panel-body > .datetime {
margin-right: 2em; }
/* line 390, static/base.scss */
body.detail main .panel-body > .btn {
clear: both;
display: block;
margin-top: 0; }
/* line 400, static/base.scss */
body.nav-active {
overflow: hidden;
position: fixed; }
/* line 404, static/base.scss */
body.nav-active #ShadowBox {
display: block; }
/* line 408, static/base.scss */
body.nav-active nav {
text-align: right;
max-height: 50px; }
/* line 412, static/base.scss */
body.nav-active nav > .container-fluid {
display: inline-block;
top: 50px;
right: 0;
width: 200px;
height: 100vh;
overflow: hidden;
position: inherit;
transform: translate(0, 0); }
/* line 422, static/base.scss */
body.nav-active nav > .container-fluid ul li {
padding-left: 1em;
text-align: left; } }
| {
"content_hash": "6a8c7c4cb5c51a470ab8eeac2ddcfd7c",
"timestamp": "",
"source": "github",
"line_count": 369,
"max_line_length": 195,
"avg_line_length": 31.498644986449865,
"alnum_prop": 0.6120622902865009,
"repo_name": "joshsimmons/animportantdate",
"id": "e43eb4e7a29882d3070e86ac07b0d9f0eef46776",
"size": "11623",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "animportantdate/staticfiles/CACHE/css/6a8c7c4cb5c5.css",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "CSS",
"bytes": "1198258"
},
{
"name": "HTML",
"bytes": "22119"
},
{
"name": "JavaScript",
"bytes": "552"
},
{
"name": "Python",
"bytes": "34198"
}
],
"symlink_target": ""
} |
package com.spotify.helios.master.resources;
import com.google.common.base.Optional;
import com.google.common.collect.Maps;
import com.codahale.metrics.annotation.ExceptionMetered;
import com.codahale.metrics.annotation.Timed;
import com.spotify.helios.common.descriptors.Deployment;
import com.spotify.helios.common.descriptors.HostStatus;
import com.spotify.helios.common.descriptors.JobId;
import com.spotify.helios.common.protocol.HostDeregisterResponse;
import com.spotify.helios.common.protocol.HostRegisterResponse;
import com.spotify.helios.common.protocol.JobDeployResponse;
import com.spotify.helios.common.protocol.JobUndeployResponse;
import com.spotify.helios.common.protocol.SetGoalResponse;
import com.spotify.helios.master.HostNotFoundException;
import com.spotify.helios.master.HostStillInUseException;
import com.spotify.helios.master.JobAlreadyDeployedException;
import com.spotify.helios.master.JobDoesNotExistException;
import com.spotify.helios.master.JobNotDeployedException;
import com.spotify.helios.master.JobPortAllocationConflictException;
import com.spotify.helios.master.MasterModel;
import com.spotify.helios.master.TokenVerificationException;
import com.spotify.helios.master.http.PATCH;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.List;
import java.util.Map;
import javax.validation.Valid;
import javax.ws.rs.DELETE;
import javax.ws.rs.DefaultValue;
import javax.ws.rs.GET;
import javax.ws.rs.POST;
import javax.ws.rs.PUT;
import javax.ws.rs.Path;
import javax.ws.rs.PathParam;
import javax.ws.rs.Produces;
import javax.ws.rs.QueryParam;
import javax.ws.rs.core.Response;
import static com.google.common.base.Strings.isNullOrEmpty;
import static com.spotify.helios.common.descriptors.Job.EMPTY_TOKEN;
import static com.spotify.helios.common.protocol.JobUndeployResponse.Status.FORBIDDEN;
import static com.spotify.helios.common.protocol.JobUndeployResponse.Status.HOST_NOT_FOUND;
import static com.spotify.helios.common.protocol.JobUndeployResponse.Status.INVALID_ID;
import static com.spotify.helios.common.protocol.JobUndeployResponse.Status.JOB_NOT_FOUND;
import static com.spotify.helios.common.protocol.JobUndeployResponse.Status.OK;
import static com.spotify.helios.master.http.Responses.badRequest;
import static com.spotify.helios.master.http.Responses.forbidden;
import static com.spotify.helios.master.http.Responses.notFound;
import static javax.ws.rs.core.MediaType.APPLICATION_JSON;
@Path("/hosts")
public class HostsResource {
private static final Logger log = LoggerFactory.getLogger(HostsResource.class);
private final MasterModel model;
public HostsResource(final MasterModel model) {
this.model = model;
}
/**
* Returns the list of hostnames of known hosts/agents.
* @return The list of hostnames.
*/
@GET
@Produces(APPLICATION_JSON)
@Timed
@ExceptionMetered
public List<String> list() {
return model.listHosts();
}
/**
* Registers a host with the cluster. The {@code host} is the name of the host. It SHOULD be
* the hostname of the machine. The {@code id} should be a persistent value for the host, but
* initially randomly generated. This way we don't have two machines claiming to be the same
* host: at least by accident.
* @param host The host to register.
* @param id The randomly generated ID for the host.
* @return The response.
*/
@PUT
@Path("{host}")
@Produces(APPLICATION_JSON)
@Timed
@ExceptionMetered
public Response.Status put(@PathParam("host") final String host,
@QueryParam("id") @DefaultValue("") final String id) {
if (isNullOrEmpty(id)) {
throw badRequest(new HostRegisterResponse(HostRegisterResponse.Status.INVALID_ID, host));
}
model.registerHost(host, id);
log.info("added host {}", host);
return Response.Status.OK;
}
/**
* Deregisters the host from the cluster. Will delete just about everything the cluster knows
* about it.
* @param host The host to deregister.
* @return The response.
*/
@DELETE
@Path("{id}")
@Produces(APPLICATION_JSON)
@Timed
@ExceptionMetered
public HostDeregisterResponse delete(@PathParam("id") final String host) {
try {
model.deregisterHost(host);
return new HostDeregisterResponse(HostDeregisterResponse.Status.OK, host);
} catch (HostNotFoundException e) {
throw notFound(new HostDeregisterResponse(HostDeregisterResponse.Status.NOT_FOUND, host));
} catch (HostStillInUseException e) {
throw badRequest(new HostDeregisterResponse(HostDeregisterResponse.Status.JOBS_STILL_DEPLOYED,
host));
}
}
/**
* Returns various status information about the host.
* @param host The host id.
* @param statusFilter An optional status filter.
* @return The host status.
*/
@GET
@Path("{id}/status")
@Produces(APPLICATION_JSON)
@Timed
@ExceptionMetered
public Optional<HostStatus> hostStatus(
@PathParam("id") final String host,
@QueryParam("status") @DefaultValue("") final String statusFilter) {
final HostStatus status = model.getHostStatus(host);
if (status != null &&
(isNullOrEmpty(statusFilter) || statusFilter.equals(status.getStatus().toString()))) {
return Optional.of(status);
} else {
return Optional.absent();
}
}
/**
* Returns various status information about the hosts.
* @param hosts The hosts.
* @param statusFilter An optional status filter.
* @return The response.
*/
@POST
@Path("/statuses")
@Produces(APPLICATION_JSON)
@Timed
@ExceptionMetered
public Map<String, HostStatus> hostStatuses(
final List<String> hosts,
@QueryParam("status") @DefaultValue("") final String statusFilter) {
final Map<String, HostStatus> statuses = Maps.newHashMap();
for (final String current : hosts) {
final HostStatus status = model.getHostStatus(current);
if (status != null) {
if (isNullOrEmpty(statusFilter) || statusFilter.equals(status.getStatus().toString())) {
statuses.put(current, status);
}
}
}
return statuses;
}
/**
* Sets the deployment of the job identified by its {@link JobId} on the host named by
* {@code host} to {@code deployment}.
* @param host The host to deploy to.
* @param jobId The job to deploy.
* @param deployment Deployment information.
* @param username The user deploying.
* @param token The authorization token for this deployment.
* @return The response.
*/
@PUT
@Path("/{host}/jobs/{job}")
@Produces(APPLICATION_JSON)
@Timed
@ExceptionMetered
public JobDeployResponse jobPut(
@PathParam("host") final String host,
@PathParam("job") final JobId jobId,
@Valid final Deployment deployment,
@RequestUser final String username,
@QueryParam("token") @DefaultValue(EMPTY_TOKEN) final String token) {
if (!jobId.isFullyQualified()) {
throw badRequest(new JobDeployResponse(JobDeployResponse.Status.INVALID_ID, host, jobId));
}
try {
final Deployment actualDeployment = deployment.toBuilder().setDeployerUser(username).build();
model.deployJob(host, actualDeployment, token);
return new JobDeployResponse(JobDeployResponse.Status.OK, host, jobId);
} catch (JobAlreadyDeployedException e) {
throw badRequest(new JobDeployResponse(JobDeployResponse.Status.JOB_ALREADY_DEPLOYED, host,
jobId));
} catch (HostNotFoundException e) {
throw badRequest(new JobDeployResponse(JobDeployResponse.Status.HOST_NOT_FOUND, host, jobId));
} catch (JobDoesNotExistException e) {
throw badRequest(new JobDeployResponse(JobDeployResponse.Status.JOB_NOT_FOUND, host, jobId));
} catch (JobPortAllocationConflictException e) {
throw badRequest(new JobDeployResponse(JobDeployResponse.Status.PORT_CONFLICT, host, jobId));
} catch (TokenVerificationException e) {
throw forbidden(new JobDeployResponse(JobDeployResponse.Status.FORBIDDEN, host, jobId));
}
}
/**
* Causes the job identified by its {@link JobId} to be undeployed from the specified host.
* This call will fail if the host is not found or the job is not deployed on the host.
* @param host The host to undeploy from.
* @param jobId The job to undeploy.
* @param token The authorization token.
* @return The response.
*/
@DELETE
@Path("/{host}/jobs/{job}")
@Produces(APPLICATION_JSON)
@Timed
@ExceptionMetered
public JobUndeployResponse jobDelete(@PathParam("host") final String host,
@PathParam("job") final JobId jobId,
@QueryParam("token") @DefaultValue("") final String token) {
if (!jobId.isFullyQualified()) {
throw badRequest(new JobUndeployResponse(INVALID_ID, host, jobId));
}
try {
model.undeployJob(host, jobId, token);
return new JobUndeployResponse(OK, host, jobId);
} catch (HostNotFoundException e) {
throw notFound(new JobUndeployResponse(HOST_NOT_FOUND, host, jobId));
} catch (JobNotDeployedException e) {
throw notFound(new JobUndeployResponse(JOB_NOT_FOUND, host, jobId));
} catch (TokenVerificationException e) {
throw forbidden(new JobUndeployResponse(FORBIDDEN, host, jobId));
}
}
/**
* Alters the current deployment of a deployed job identified by it's job id on the specified
* host.
* @param host The host.
* @param jobId The ID of the job.
* @param deployment The new deployment.
* @param token The authorization token for this job.
* @return The response.
*/
@PATCH
@Path("/{host}/jobs/{job}")
@Produces(APPLICATION_JSON)
@Timed
@ExceptionMetered
public SetGoalResponse jobPatch(@PathParam("host") final String host,
@PathParam("job") final JobId jobId,
@Valid final Deployment deployment,
@QueryParam("token") @DefaultValue("") final String token) {
if (!deployment.getJobId().equals(jobId)) {
throw badRequest(new SetGoalResponse(SetGoalResponse.Status.ID_MISMATCH, host, jobId));
}
try {
model.updateDeployment(host, deployment, token);
} catch (HostNotFoundException e) {
throw notFound(new SetGoalResponse(SetGoalResponse.Status.HOST_NOT_FOUND, host, jobId));
} catch (JobNotDeployedException e) {
throw notFound(new SetGoalResponse(SetGoalResponse.Status.JOB_NOT_DEPLOYED, host, jobId));
} catch (TokenVerificationException e) {
throw forbidden(new SetGoalResponse(SetGoalResponse.Status.FORBIDDEN, host, jobId));
}
log.info("patched job {} on host {}", deployment, host);
return new SetGoalResponse(SetGoalResponse.Status.OK, host, jobId);
}
/**
* Returns the current {@link Deployment} of {@code job} on {@code host} if it is deployed.
* @param host The host where the job is deployed.
* @param jobId The ID of the job.
* @return The response.
*/
@GET
@Path("/{host}/jobs/{job}")
@Produces(APPLICATION_JSON)
@Timed
@ExceptionMetered
public Optional<Deployment> jobGet(@PathParam("host") final String host,
@PathParam("job") final JobId jobId) {
if (!jobId.isFullyQualified()) {
throw badRequest();
}
return Optional.fromNullable(model.getDeployment(host, jobId));
}
}
| {
"content_hash": "945b9ba2bceb7b39653c9ec0b20986eb",
"timestamp": "",
"source": "github",
"line_count": 303,
"max_line_length": 100,
"avg_line_length": 38.06270627062706,
"alnum_prop": 0.7051937917280846,
"repo_name": "gtonic/helios",
"id": "f98e2eb49bbb204b4cd6e1197a0a6ea26f2041c8",
"size": "12133",
"binary": false,
"copies": "2",
"ref": "refs/heads/master",
"path": "helios-services/src/main/java/com/spotify/helios/master/resources/HostsResource.java",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "Java",
"bytes": "2267506"
},
{
"name": "Makefile",
"bytes": "269"
},
{
"name": "Python",
"bytes": "5057"
},
{
"name": "Shell",
"bytes": "27739"
}
],
"symlink_target": ""
} |
cd ../SWFs/Assets
$SDK_DIR/bin/mxmlc -includes=mx.managers.systemClasses.MarshallingSupport -source-path=../../../../../as3/src/mustella -includes=UnitTester -theme=$SDK_DIR/frameworks/themes/Halo/halo.swc child_swfs/MP_Alert_Child.mxml | {
"content_hash": "1276332db3102d075fbd83b7a3fdca52",
"timestamp": "",
"source": "github",
"line_count": 3,
"max_line_length": 218,
"avg_line_length": 79,
"alnum_prop": 0.759493670886076,
"repo_name": "shyamalschandra/flex-sdk",
"id": "a4c0ecbae4c6278fffc00e5561f2556ab23aa134",
"size": "1222",
"binary": false,
"copies": "10",
"ref": "refs/heads/master",
"path": "mustella/tests/components/Alert/Versioning/MP_Alert_Tests.sh",
"mode": "33261",
"license": "apache-2.0",
"language": [
{
"name": "AGS Script",
"bytes": "478"
},
{
"name": "ASP",
"bytes": "6381"
},
{
"name": "ActionScript",
"bytes": "34905828"
},
{
"name": "Awk",
"bytes": "1958"
},
{
"name": "Batchfile",
"bytes": "40336"
},
{
"name": "C",
"bytes": "4601"
},
{
"name": "C++",
"bytes": "10259"
},
{
"name": "CSS",
"bytes": "508448"
},
{
"name": "Groff",
"bytes": "59633"
},
{
"name": "HTML",
"bytes": "84174"
},
{
"name": "Java",
"bytes": "15072097"
},
{
"name": "JavaScript",
"bytes": "110516"
},
{
"name": "PureBasic",
"bytes": "362"
},
{
"name": "Shell",
"bytes": "306431"
},
{
"name": "Visual Basic",
"bytes": "4498"
},
{
"name": "XSLT",
"bytes": "757371"
}
],
"symlink_target": ""
} |
/**
* This is Free Software. See COPYING for information.
*/
#ifndef _PUZZLE_SELECTION_RENDERER_INTERFACE_HPP_
#define _PUZZLE_SELECTION_RENDERER_INTERFACE_HPP_
#include "renderer_interface.hpp"
#include <string>
/**
* Class that knows how to render a list of puzzles for selection
*/
class PuzzleSelectionRendererInterface :
public RendererInterface
{
public:
PuzzleSelectionRendererInterface() :
RendererInterface() {
}
virtual ~PuzzleSelectionRendererInterface() {
}
virtual void render_puzzle_name(std::string & i_name, int i_index) = 0;
virtual int get_mouse_position_as_puzzle_index(int i_x, int i_y) = 0;
virtual void highlight_puzzle_name(int i_index) = 0;
};
#endif // _PUZZLE_SELECTION_RENDERER_INTERFACE_HPP_ | {
"content_hash": "e864e6a5805c27f7571ef57bf79ec459",
"timestamp": "",
"source": "github",
"line_count": 35,
"max_line_length": 73,
"avg_line_length": 21.62857142857143,
"alnum_prop": 0.726552179656539,
"repo_name": "phtrivier/ube",
"id": "89f37c98f3265615a215c36c5d25fb0218772c66",
"size": "757",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "src/client/puzzle_selection_renderer_interface.hpp",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "C",
"bytes": "359703"
},
{
"name": "C++",
"bytes": "1581336"
},
{
"name": "Emacs Lisp",
"bytes": "2410"
},
{
"name": "Lua",
"bytes": "33216"
},
{
"name": "Python",
"bytes": "81906"
},
{
"name": "Ruby",
"bytes": "3247"
},
{
"name": "Shell",
"bytes": "6925"
}
],
"symlink_target": ""
} |
<html>
<head>
<title>Embedding JS demonstration</title>
</head>
<body>
<input type="text" id="dataSetId" value="4" placeholder="DataSet id">
<input type="button" id="loadDataSet" value="loadDataSet">
Force switch reference:
<input type="checkbox" id="forceSwitchRefDataSet">
<p>
<input type="text" id="coordinatesText" value="" placeholder="chromosome: coordinates">
<input type="button" id="navigateToCoordinate" value="navigateToCoordinate">
<p>
<input type="button" id="toggleSelectTrackId" value="toggleSelectTrack (by id)">
<input type="button" id="toggleSelectTrackUrl" value="toggleSelectTrack (by Url)">
<input type="button" id="loadTrackUrl" value="loadTrack (Url)">
<input type="button" id="loadTrackNgb" value="loadTrack (path on ngbServer)">
Force switch reference:
<input type="checkbox" id="forceSwitchRefTrack">
<p>
<p>
<textarea rows="10" cols="35" id="globalSettings"></textarea>
<input type="button" id="setGlobalSettings" value="setGlobalSettings">
<textarea rows="10" cols="35" id="trackSettings"></textarea>
<select name="typeTrack" id="typeTrack">
<option value="vcf">vcf</option>
<option value="referense">referense</option>
<option value="wig">wig</option>
<option value="bam">bam</option>
<option value="gene">gene</option>
</select>
<input type="button" id="setTrackSettings" value="setTrackSettings">
</p>
<p>
<input type="text" id="token" value="" placeholder="token">
<input type="button" id="setToken" value="setToken">
</p>
<iframe height="90%" width="100%" id="ngbIframe" src="http://localhost:8080/#"></iframe>
<script type="text/javascript">
var dataSetId = document.getElementById('dataSetId'),
forceSwitchRefDataSet = document.getElementById('forceSwitchRefDataSet'),
btnLoadDataSet = document.getElementById('loadDataSet'),
btnNavigateToCoordinate = document.getElementById('navigateToCoordinate'),
coordinatesInput = document.getElementById('coordinatesText'),
btnToggleSelectTrackId = document.getElementById('toggleSelectTrackId'),
btnLoadTrackUrl = document.getElementById('loadTrackUrl'),
btnLoadTrackNgb = document.getElementById('loadTrackNgb'),
btnToggleSelectTrackUrl = document.getElementById('toggleSelectTrackUrl'),
forceSwitchRefTrack = document.getElementById('forceSwitchRefTrack'),
globalSettings = document.getElementById('globalSettings'),
btnSetGlobalSettings = document.getElementById('setGlobalSettings'),
trackSettings = document.getElementById('trackSettings'),
typeTrackSelect = document.getElementById('typeTrack'),
btnSetTrackSettings = document.getElementById('setTrackSettings'),
token = document.getElementById('token'),
btnSetToken = document.getElementById('setToken'),
iframe = document.getElementById('ngbIframe');
init();
btnLoadDataSet.onclick = function () {
iframe.contentWindow.postMessage({
callerId: guid(),
method: "loadDataSet",
params: {
id: dataSetId.value,
forceSwitchRef: forceSwitchRefDataSet.checked,
}
}, "*");
};
btnNavigateToCoordinate.onclick = function () {
iframe.contentWindow.postMessage({
callerId: guid(),
method: "navigateToCoordinate",
params: {coordinates: coordinatesInput.value}
}, "*");
};
btnToggleSelectTrackId.onclick = function () {
iframe.contentWindow.postMessage({
callerId: guid(),
method: "toggleSelectTrack",
params: {
track: 21,
forceSwitchRef: forceSwitchRefTrack.checked,
}
}, "*");
};
btnToggleSelectTrackUrl.onclick = function () {
iframe.contentWindow.postMessage({
callerId: guid(),
method: "toggleSelectTrack",
params: {
track: "http://ngb.opensource.epam.com/distr/data/demo/ngb_demo_data/sample_1-lumpy.vcf.gz",
forceSwitchRef: forceSwitchRefTrack.checked,
}
}, "*");
};
btnLoadTrackUrl.onclick = function () {
iframe.contentWindow.postMessage({
callerId: guid(),
method: "loadTracks",
params: {
tracks: [{
path: "http://ngb.opensource.epam.com/distr/data/demo/ngb_demo_data/sample_1-lumpy.vcf.gz",
index: "http://ngb.opensource.epam.com/distr/data/demo/ngb_demo_data/sample_1-lumpy.vcf.gz.tbi",
}, {
path: "http://ngb.opensource.epam.com/distr/data/genome/dm6/dmel-all-r6.06.sorted.gtf.gz",
index: "http://ngb.opensource.epam.com/distr/data/genome/dm6/dmel-all-r6.06.sorted.gtf.gz.tbi",
}, {
path: "http://ngb.opensource.epam.com/distr/data/genome/grch38/Homo_sapiens.GRCh38.gff3.gz",
index: "http://ngb.opensource.epam.com/distr/data/genome/grch38/Homo_sapiens.GRCh38.gff3.gz.tbi",
}, {
path: "http://ngb.opensource.epam.com/distr/data/demo/ngb_demo_data/PIK3CA-E545K.bam",
index: "http://ngb.opensource.epam.com/distr/data/demo/ngb_demo_data/PIK3CA-E545K.bam.bai",
}, {
path: "http://ngb.opensource.epam.com/distr/data/genome/grch38/Homo_sapiens.GRCh38.domains.bed.gz",
index: "http://ngb.opensource.epam.com/distr/data/genome/grch38/Homo_sapiens.GRCh38.domains.bed.gz.tbi",
},
],
forceSwitchRef: forceSwitchRefTrack.checked,
referenceId: 3,
mode: 'url',
}
}, "*");
};
btnLoadTrackNgb.onclick = function () {
iframe.contentWindow.postMessage({
callerId: guid(),
method: "loadTracks",
params: {
tracks: [{
path: "/opt/catgenome/MCF7-PIK3CA.bam",
index: "/opt/catgenome/MCF7-PIK3CA.bam.bai",
}, {
path: "/opt/catgenome/beds-test/1.bed.gz",
index: "/opt/catgenome/beds-test/1.bed.gz.tbi",
}, {
path: "/opt/catgenome/beds-test/2.bed.gz",
index: "/opt/catgenome/beds-test/2.bed.gz.tbi",
}, {
path: "/opt/catgenome/beds-test/3.bed.gz",
index: "/opt/catgenome/beds-test/3.bed.gz.tbi",
}, {
path: "/opt/catgenome/beds-test/BRD4_primers_names.bed.gz",
index: "/opt/catgenome/beds-test/BRD4_primers_names.bed.gz.tbi",
},
],
forceSwitchRef: forceSwitchRefTrack.checked,
referenceId: 1,
mode: 'ngbServer',
}
}, "*");
};
btnSetGlobalSettings.onclick = function () {
iframe.contentWindow.postMessage({
callerId: guid(),
method: "setGlobalSettings",
params: globalSettings.value ? JSON.parse(globalSettings.value) : null
}, "*");
};
btnSetTrackSettings.onclick = function () {
iframe.contentWindow.postMessage({
callerId: guid(),
method: "setTrackSettings",
params: trackSettings.value ? JSON.parse(trackSettings.value) : null
}, "*");
};
btnSetToken.onclick = function () {
iframe.contentWindow.postMessage({
callerId: guid(),
method: "setToken",
params: {token: token.value}
}, "*");
}
typeTrackSelect.onchange = function () {
switch (typeTrackSelect.value) {
case 'vcf':
trackSettings.value = JSON.stringify({
id: 23, // id or path "http://ngb.opensource.epam.com/distr/data/demo/ngb_demo_data/sample_1-lumpy.vcf.gz"
settings: [
{name: "vcf>variantsView>expanded"}
]
});
break;
case 'referense':
trackSettings.value = JSON.stringify({
id: 5,
settings: [
{name: "reference>showTranslation", value: true},
{name: "reference>showForwardStrand", value: true},
{name: "reference>showReverseStrand", value: true}
]
});
break;
case 'wig':
trackSettings.value = JSON.stringify({
id: 28,
settings: [
{
name: "coverage>scale>manual", value: true, extraOptions: {
from: 10,
to: 150
}
},
{name: "coverage>scale>log", value: true}
]
});
break;
case 'bam':
trackSettings.value = JSON.stringify({
id: 43,
settings: [
{name: "bam>color>firstInPairStrand", value: true},
{name: "bam>color>shadeByQuality", value: true},
{name: "bam>group>firstInPairStrand", value: true},
{name: "bam>readsView>expanded"},
{name: "bam>readsView>pairs", value: true},
{name: "bam>sort>mappingQuality"},
{name: "bam>showAlignments", value: true},
{name: "bam>showMismatchedBases", value: true},
{name: "bam>showCoverage", value: true},
{name: "bam>showSpliceJunctions", value: true},
{name: "coverage>scale>default", value: false},
{
name: "coverage>scale>manual", value: true, extraOptions: {
from: 10,
to: 150
}
},
{name: "coverage>scale>log", value: true}
]
});
break;
case 'gene':
trackSettings.value = JSON.stringify({
id: 59,
settings: [
{name: "gene>transcript>expanded"},
{name: "shortenIntrons", value: true}
]
});
break;
}
};
function guid() {
function s4() {
return Math.floor((1 + Math.random()) * 0x10000)
.toString(16)
.substring(1);
}
return s4() + s4() + '-' + s4() + '-' + s4() + '-' +
s4() + '-' + s4() + s4() + s4();
}
function init() {
globalSettings.value = JSON.stringify({
showCenterLine: false,
hotkeys: {
"layout>variants": {
"hotkey": "ALT + !"
},
'gene>transcript>expanded': {
"hotkey": "ALT + $"
}
},
colors: {
strand: {forward: 14363949}
}
});
trackSettings.value = JSON.stringify({
id: 23, // id or path like "http://ngb.opensource.epam.com/distr/data/demo/ngb_demo_data/sample_1-lumpy.vcf.gz"
settings: [
{name: "vcf>variantsView>expanded"}
]
});
}
if (window.addEventListener) {
window.addEventListener("message", () => {
if (event.data) {
console.log(event.data);
}
});
}
</script>
</body>
</html>
| {
"content_hash": "e13d91844c6f000b4ddd8d0a0ae06f9d",
"timestamp": "",
"source": "github",
"line_count": 309,
"max_line_length": 126,
"avg_line_length": 39.006472491909385,
"alnum_prop": 0.5149755247656185,
"repo_name": "epam/NGB",
"id": "574b66a88a06eb343b5bac157ce743e6dfd077c3",
"size": "12053",
"binary": false,
"copies": "1",
"ref": "refs/heads/develop",
"path": "docs/md/user-guide/embedding-js-demo.html",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "CSS",
"bytes": "2130"
},
{
"name": "Dockerfile",
"bytes": "2839"
},
{
"name": "EJS",
"bytes": "344"
},
{
"name": "HTML",
"bytes": "455953"
},
{
"name": "Java",
"bytes": "6041257"
},
{
"name": "JavaScript",
"bytes": "3315081"
},
{
"name": "PLSQL",
"bytes": "1182"
},
{
"name": "SCSS",
"bytes": "167986"
},
{
"name": "Shell",
"bytes": "11389"
},
{
"name": "Smarty",
"bytes": "1410"
}
],
"symlink_target": ""
} |
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml" lang="" xml:lang="">
<head>
<meta charset="utf-8" />
<meta name="generator" content="pandoc" />
<meta name="viewport" content="width=device-width, initial-scale=1.0, user-scalable=yes" />
<meta name="author" content="" />
<meta name="dcterms.date" content="2021-10-27" />
<title>XMONAD(1) Tiling Window Manager</title>
<style type="text/css">
code{white-space: pre-wrap;}
span.smallcaps{font-variant: small-caps;}
span.underline{text-decoration: underline;}
div.column{display: inline-block; vertical-align: top; width: 50%;}
</style>
<style type="text/css">
a.sourceLine { display: inline-block; line-height: 1.25; }
a.sourceLine { pointer-events: none; color: inherit; text-decoration: inherit; }
a.sourceLine:empty { height: 1.2em; }
.sourceCode { overflow: visible; }
code.sourceCode { white-space: pre; position: relative; }
div.sourceCode { margin: 1em 0; }
pre.sourceCode { margin: 0; }
@media screen {
div.sourceCode { overflow: auto; }
}
@media print {
code.sourceCode { white-space: pre-wrap; }
a.sourceLine { text-indent: -1em; padding-left: 1em; }
}
pre.numberSource a.sourceLine
{ position: relative; left: -4em; }
pre.numberSource a.sourceLine::before
{ content: attr(title);
position: relative; left: -1em; text-align: right; vertical-align: baseline;
border: none; pointer-events: all; display: inline-block;
-webkit-touch-callout: none; -webkit-user-select: none;
-khtml-user-select: none; -moz-user-select: none;
-ms-user-select: none; user-select: none;
padding: 0 4px; width: 4em;
color: #aaaaaa;
}
pre.numberSource { margin-left: 3em; border-left: 1px solid #aaaaaa; padding-left: 4px; }
div.sourceCode
{ }
@media screen {
a.sourceLine::before { text-decoration: underline; }
}
code span.al { color: #ff0000; font-weight: bold; } /* Alert */
code span.an { color: #60a0b0; font-weight: bold; font-style: italic; } /* Annotation */
code span.at { color: #7d9029; } /* Attribute */
code span.bn { color: #40a070; } /* BaseN */
code span.bu { } /* BuiltIn */
code span.cf { color: #007020; font-weight: bold; } /* ControlFlow */
code span.ch { color: #4070a0; } /* Char */
code span.cn { color: #880000; } /* Constant */
code span.co { color: #60a0b0; font-style: italic; } /* Comment */
code span.cv { color: #60a0b0; font-weight: bold; font-style: italic; } /* CommentVar */
code span.do { color: #ba2121; font-style: italic; } /* Documentation */
code span.dt { color: #902000; } /* DataType */
code span.dv { color: #40a070; } /* DecVal */
code span.er { color: #ff0000; font-weight: bold; } /* Error */
code span.ex { } /* Extension */
code span.fl { color: #40a070; } /* Float */
code span.fu { color: #06287e; } /* Function */
code span.im { } /* Import */
code span.in { color: #60a0b0; font-weight: bold; font-style: italic; } /* Information */
code span.kw { color: #007020; font-weight: bold; } /* Keyword */
code span.op { color: #666666; } /* Operator */
code span.ot { color: #007020; } /* Other */
code span.pp { color: #bc7a00; } /* Preprocessor */
code span.sc { color: #4070a0; } /* SpecialChar */
code span.ss { color: #bb6688; } /* SpecialString */
code span.st { color: #4070a0; } /* String */
code span.va { color: #19177c; } /* Variable */
code span.vs { color: #4070a0; } /* VerbatimString */
code span.wa { color: #60a0b0; font-weight: bold; font-style: italic; } /* Warning */
</style>
</head>
<body>
<header id="title-block-header">
<h1 class="title">XMONAD(1) Tiling Window Manager</h1>
<p class="author"></p>
<p class="date">27 October 2021</p>
</header>
<nav id="TOC">
<ul>
<li><a href="#name">Name</a></li>
<li><a href="#description">Description</a></li>
<li><a href="#usage">Usage</a><ul>
<li><a href="#flags">Flags</a></li>
<li><a href="#default-keyboard-bindings">Default keyboard bindings</a></li>
</ul></li>
<li><a href="#examples">Examples</a></li>
<li><a href="#customization">Customization</a><ul>
<li><a href="#modular-configuration">Modular Configuration</a></li>
</ul></li>
<li><a href="#bugs">Bugs</a></li>
</ul>
</nav>
<h1 id="name">Name</h1>
<p>xmonad - Tiling Window Manager</p>
<h1 id="description">Description</h1>
<p><em>xmonad</em> is a minimalist tiling window manager for X, written in Haskell. Windows are managed using automatic layout algorithms, which can be dynamically reconfigured. At any time windows are arranged so as to maximize the use of screen real estate. All features of the window manager are accessible purely from the keyboard: a mouse is entirely optional. <em>xmonad</em> is configured in Haskell, and custom layout algorithms may be implemented by the user in config files. A principle of <em>xmonad</em> is predictability: the user should know in advance precisely the window arrangement that will result from any action.</p>
<p>By default, <em>xmonad</em> provides three layout algorithms: tall, wide and fullscreen. In tall or wide mode, windows are tiled and arranged to prevent overlap and maximize screen use. Sets of windows are grouped together on virtual screens, and each screen retains its own layout, which may be reconfigured dynamically. Multiple physical monitors are supported via Xinerama, allowing simultaneous display of a number of screens.</p>
<p>By utilizing the expressivity of a modern functional language with a rich static type system, <em>xmonad</em> provides a complete, featureful window manager in less than 1200 lines of code, with an emphasis on correctness and robustness. Internal properties of the window manager are checked using a combination of static guarantees provided by the type system, and type-based automated testing. A benefit of this is that the code is simple to understand, and easy to modify.</p>
<h1 id="usage">Usage</h1>
<p><em>xmonad</em> places each window into a “workspace”. Each workspace can have any number of windows, which you can cycle though with mod-j and mod-k. Windows are either displayed full screen, tiled horizontally, or tiled vertically. You can toggle the layout mode with mod-space, which will cycle through the available modes.</p>
<p>You can switch to workspace N with mod-N. For example, to switch to workspace 5, you would press mod-5. Similarly, you can move the current window to another workspace with mod-shift-N.</p>
<p>When running with multiple monitors (Xinerama), each screen has exactly 1 workspace visible. mod-{w,e,r} switch the focus between screens, while shift-mod-{w,e,r} move the current window to that screen. When <em>xmonad</em> starts, workspace 1 is on screen 1, workspace 2 is on screen 2, etc. When switching workspaces to one that is already visible, the current and visible workspaces are swapped.</p>
<h2 id="flags">Flags</h2>
<p>xmonad has several flags which you may pass to the executable. These flags are:</p>
<dl>
<dt>–recompile</dt>
<dd>Recompiles your <em>xmonad.hs</em> configuration
</dd>
<dt>–restart</dt>
<dd>Causes the currently running <em>xmonad</em> process to restart
</dd>
<dt>–replace</dt>
<dd>Replace the current window manager with xmonad
</dd>
<dt>–version</dt>
<dd>Display version of <em>xmonad</em>
</dd>
<dt>–verbose-version</dt>
<dd>Display detailed version of <em>xmonad</em>
</dd>
</dl>
<h2 id="default-keyboard-bindings">Default keyboard bindings</h2>
<dl>
<dt>mod-shift-return</dt>
<dd>Launch terminal
</dd>
<dt>mod-p</dt>
<dd>Launch dmenu
</dd>
<dt>mod-shift-p</dt>
<dd>Launch gmrun
</dd>
<dt>mod-shift-c</dt>
<dd>Close the focused window
</dd>
<dt>mod-space</dt>
<dd>Rotate through the available layout algorithms
</dd>
<dt>mod-shift-space</dt>
<dd>Reset the layouts on the current workspace to default
</dd>
<dt>mod-n</dt>
<dd>Resize viewed windows to the correct size
</dd>
<dt>mod-tab</dt>
<dd>Move focus to the next window
</dd>
<dt>mod-shift-tab</dt>
<dd>Move focus to the previous window
</dd>
<dt>mod-j</dt>
<dd>Move focus to the next window
</dd>
<dt>mod-k</dt>
<dd>Move focus to the previous window
</dd>
<dt>mod-m</dt>
<dd>Move focus to the master window
</dd>
<dt>mod-return</dt>
<dd>Swap the focused window and the master window
</dd>
<dt>mod-shift-j</dt>
<dd>Swap the focused window with the next window
</dd>
<dt>mod-shift-k</dt>
<dd>Swap the focused window with the previous window
</dd>
<dt>mod-h</dt>
<dd>Shrink the master area
</dd>
<dt>mod-l</dt>
<dd>Expand the master area
</dd>
<dt>mod-t</dt>
<dd>Push window back into tiling
</dd>
<dt>mod-comma</dt>
<dd>Increment the number of windows in the master area
</dd>
<dt>mod-period</dt>
<dd>Deincrement the number of windows in the master area
</dd>
<dt>mod-shift-q</dt>
<dd>Quit xmonad
</dd>
<dt>mod-q</dt>
<dd>Restart xmonad
</dd>
<dt>mod-shift-slash</dt>
<dd>Run xmessage with a summary of the default keybindings (useful for beginners)
</dd>
<dt>mod-question</dt>
<dd>Run xmessage with a summary of the default keybindings (useful for beginners)
</dd>
<dt>mod-[1..9]</dt>
<dd>Switch to workspace N
</dd>
<dt>mod-shift-[1..9]</dt>
<dd>Move client to workspace N
</dd>
<dt>mod-{w,e,r}</dt>
<dd>Switch to physical/Xinerama screens 1, 2, or 3
</dd>
<dt>mod-shift-{w,e,r}</dt>
<dd>Move client to screen 1, 2, or 3
</dd>
<dt>mod-button1</dt>
<dd>Set the window to floating mode and move by dragging
</dd>
<dt>mod-button2</dt>
<dd>Raise the window to the top of the stack
</dd>
<dt>mod-button3</dt>
<dd>Set the window to floating mode and resize by dragging
</dd>
</dl>
<h1 id="examples">Examples</h1>
<p>To use xmonad as your window manager add to your <em>~/.xinitrc</em> file:</p>
<blockquote>
<p>exec xmonad</p>
</blockquote>
<h1 id="customization">Customization</h1>
<p>xmonad is customized in your <em>xmonad.hs</em>, and then restarted with mod-q. You can choose where your configuration file lives by</p>
<ol type="1">
<li>Setting <code>XMONAD_DATA_DIR,</code> <code>XMONAD_CONFIG_DIR</code>, and <code>XMONAD_CACHE_DIR</code>; <em>xmonad.hs</em> is then expected to be in <code>XMONAD_CONFIG_DIR</code>.</li>
<li>Creating <em>xmonad.hs</em> in <em>~/.xmonad</em>.</li>
<li>Creating <em>xmonad.hs</em> in <code>XDG_CONFIG_HOME</code>. Note that, in this case, xmonad will use <code>XDG_DATA_HOME</code> and <code>XDG_CACHE_HOME</code> for its data and cache directory respectively.</li>
</ol>
<p>You can find many extensions to the core feature set in the xmonad- contrib package, available through your package manager or from <a href="https://xmonad.org">xmonad.org</a>.</p>
<h2 id="modular-configuration">Modular Configuration</h2>
<p>As of <em>xmonad-0.9</em>, any additional Haskell modules may be placed in <em>~/.xmonad/lib/</em> are available in GHC’s searchpath. Hierarchical modules are supported: for example, the file <em>~/.xmonad/lib/XMonad/Stack/MyAdditions.hs</em> could contain:</p>
<div class="sourceCode" id="cb1"><pre class="sourceCode haskell"><code class="sourceCode haskell"><a class="sourceLine" id="cb1-1" title="1"><span class="kw">module</span> <span class="dt">XMonad.Stack.MyAdditions</span> (function1) <span class="kw">where</span></a>
<a class="sourceLine" id="cb1-2" title="2"> function1 <span class="ot">=</span> <span class="fu">error</span> <span class="st">"function1: Not implemented yet!"</span></a></code></pre></div>
<p>Your xmonad.hs may then import XMonad.Stack.MyAdditions as if that module was contained within xmonad or xmonad-contrib.</p>
<h1 id="bugs">Bugs</h1>
<p>Probably. If you find any, please report them to the <a href="https://github.com/xmonad/xmonad/issues">bugtracker</a></p>
</body>
</html>
| {
"content_hash": "d2291b0e2daf38d119c10d134c3a449a",
"timestamp": "",
"source": "github",
"line_count": 247,
"max_line_length": 637,
"avg_line_length": 46.57085020242915,
"alnum_prop": 0.7049465356863427,
"repo_name": "xmonad/xmonad",
"id": "a5b7e2bea47489e8160229038490ff3a1306c3be",
"size": "11519",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "man/xmonad.1.html",
"mode": "33188",
"license": "bsd-3-clause",
"language": [
{
"name": "Haskell",
"bytes": "193197"
},
{
"name": "Nix",
"bytes": "3901"
},
{
"name": "Shell",
"bytes": "735"
}
],
"symlink_target": ""
} |
<?php
require_once '../app/models/User.php';
/**
* HomeController demostrative example
*/
class HomeController {
/**
* Method index
* Demostrative example of index
* @return [type] [description]
*/
public function index()
{
// Instance of View
$view = new View('home');
// add assets styles to view
$view->assets('styles',array(
'css/styles.css',
));
// add assets javascripts to view
$view->assets('javascripts',array(
'js/custom.js',
));
// add one var to View
$view->data('author','Dav Lizárraga');
// Intance of User
$user = new User();
// add user to DB.
$user->addUser('David','[email protected]');
// add array data to View
$view->dataArray('numeros',array('uno','dos','tres','cuatro','cinco'));
// return view instance.
return $view;
}
} | {
"content_hash": "4daac9d0b4c40a46fb93e247527b4cdb",
"timestamp": "",
"source": "github",
"line_count": 46,
"max_line_length": 76,
"avg_line_length": 20.934782608695652,
"alnum_prop": 0.5202492211838006,
"repo_name": "davgeek/Muffinphp",
"id": "528aa6e3a5f25f1e9ed23ab739c0e3b52bf54b5d",
"size": "964",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "app/controllers/HomeController.php",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "ApacheConf",
"bytes": "145"
},
{
"name": "CSS",
"bytes": "88"
},
{
"name": "PHP",
"bytes": "14825"
}
],
"symlink_target": ""
} |
'use strict';
var util = require('util');
var _ = require('lodash');
var yeoman = require('yeoman-generator');
var PlayBase = require('../utils/play-base');
var SnippetGenerator = module.exports = function SnippetGenerator(args, options, config) {
PlayBase.apply(this, arguments);
this.argument("snippetName", {
required: true,
optional: false,
type: "String"
});
this.option("path", {
type: "String"
});
};
util.inherits(SnippetGenerator, PlayBase);
SnippetGenerator.prototype.askForSnippet = function () {
var snippetPath = this.options.path ? this.options.path + "/" + this.snippetName : this.sourceRoot() + "/" + this.snippetName;
this.snippet = require(snippetPath);
if (!this.snippet) {
console.log("No snippet at ", snippetPath);
} else {
if (this.snippet.prompts) {
var cb = this.async();
this.prompt(this.snippet.prompts, function (props, err) {
if (err) {
return this.emit('error', err);
}
this.snippet = this.recursiveEngines()[this.snippet.engine || "default"].call(this, this.snippet, props);
cb();
}.bind(this));
}
}
};
SnippetGenerator.prototype.writesnippet = function () {
if (this.snippet) {
var packagePath = this.paths.root + "/package.json";
var bowerPath = this.paths.root + "/bower.json";
var gruntPath = this.paths.root + "/Gruntfile.js";
var constantsPath = this.paths.conf + "/constants.json";
this._updateJson(packagePath, "package");
this._updateJson(bowerPath, "bower");
this._updateJson(constantsPath, "constants");
if (this.snippet.grunt && this.existsFile(gruntPath)) {
var gruntfile = this.readFileAsString(gruntPath);
var startToken = "initConfig(";
var endToken = ");";
var startConfig = gruntfile.indexOf(startToken) + startToken.length;
var endConfig = gruntfile.indexOf(endToken, startConfig);
var gruntConfig = gruntfile.substring(startConfig, endConfig);
// Preserve all JavaScript variables inside the Gruntfile
var variables = ["configuration"];
var variablesMapping = {};
_.forEach(variables, function (variable) {
var stringVariable = "@{" + variable + "}";
variablesMapping[variable] = stringVariable;
gruntConfig = gruntConfig.replace(variable, "variablesMapping." + variable);
});
var gruntConfigObject;
eval("gruntConfigObject = " + gruntConfig);
gruntConfigObject = this._merge(gruntConfigObject, this.snippet.grunt);
gruntConfig = JSON.stringify(gruntConfigObject, null, " ");
_.forEach(variablesMapping, function (stringVariable, variable) {
gruntConfig = gruntConfig.replace("\"" + stringVariable + "\"", variable);
});
gruntfile = gruntfile.substring(0, startConfig) + gruntConfig + gruntfile.substring(endConfig);
this.writeFileFromString(gruntfile, "Gruntfile.js");
}
}
};
SnippetGenerator.prototype._merge = function (source1, source2) {
return _.merge(source1, source2, function (a, b) {
return _.isArray(a) ? a.concat(b) : undefined;
});
};
SnippetGenerator.prototype._updateJson = function (path, property) {
if (this.snippet[property] && this.existsFile(path)) {
var data = this.readFileAsJson(path);
data = this._merge(data, this.snippet[property]);
this.writeFileFromJson(path, data);
}
};
| {
"content_hash": "4774771e9862ccd157d4dc1a48858f81",
"timestamp": "",
"source": "github",
"line_count": 105,
"max_line_length": 128,
"avg_line_length": 32.31428571428572,
"alnum_prop": 0.65929855585028,
"repo_name": "pauldijou/generator-play",
"id": "ec8fef8cca7e59ce62924745a1fbeca3380ec1c0",
"size": "3393",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "snippet/index.js",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "CSS",
"bytes": "0"
},
{
"name": "Java",
"bytes": "2017"
},
{
"name": "JavaScript",
"bytes": "36641"
},
{
"name": "Scala",
"bytes": "3693"
}
],
"symlink_target": ""
} |
function [sys,x0,str,ts] = var_eta1_tc(t,x,u,flag)
%SFUNTMPL General M-file S-function template
% With M-file S-functions, you can define you own ordinary differential
% equations (ODEs), discrete system equations, and/or just about
% any type of algorithm to be used within a Simulink block diagram.
%
% The general form of an M-File S-function syntax is:
% [SYS,X0,STR,TS] = SFUNC(T,X,U,FLAG,P1,...,Pn)
%
% What is returned by SFUNC at a given point in time, T, depends on the
% value of the FLAG, the current state vector, X, and the current
% input vector, U.
%
% FLAG RESULT DESCRIPTION
% ----- ------ --------------------------------------------
% 0 [SIZES,X0,STR,TS] Initialization, return system sizes in SYS,
% initial state in X0, state ordering strings
% in STR, and sample times in TS.
% 1 DX Return continuous state derivatives in SYS.
% 2 DS Update discrete states SYS = X(n+1)
% 3 Y Return outputs in SYS.
% 4 TNEXT Return next time hit for variable step sample
% time in SYS.
% 5 Reserved for future (root finding).
% 9 [] Termination, perform any cleanup SYS=[].
%
%
% The state vectors, X and X0 consists of continuous states followed
% by discrete states.
%
% Optional parameters, P1,...,Pn can be provided to the S-function and
% used during any FLAG operation.
%
% When SFUNC is called with FLAG = 0, the following information
% should be returned:
%
% SYS(1) = Number of continuous states.
% SYS(2) = Number of discrete states.
% SYS(3) = Number of outputs.
% SYS(4) = Number of inputs.
% Any of the first four elements in SYS can be specified
% as -1 indicating that they are dynamically sized. The
% actual length for all other flags will be equal to the
% length of the input, U.
% SYS(5) = Reserved for root finding. Must be zero.
% SYS(6) = Direct feedthrough flag (1=yes, 0=no). The s-function
% has direct feedthrough if U is used during the FLAG=3
% call. Setting this to 0 is akin to making a promise that
% U will not be used during FLAG=3. If you break the promise
% then unpredictable results will occur.
% SYS(7) = Number of sample times. This is the number of rows in TS.
%
%
% X0 = Initial state conditions or [] if no states.
%
% STR = State ordering strings which is generally specified as [].
%
% TS = An m-by-2 matrix containing the sample time
% (period, offset) information. Where m = number of sample
% times. The ordering of the sample times must be:
%
% TS = [0 0, : Continuous sample time.
% 0 1, : Continuous, but fixed in minor step
% sample time.
% PERIOD OFFSET, : Discrete sample time where
% PERIOD > 0 & OFFSET < PERIOD.
% -2 0]; : Variable step discrete sample time
% where FLAG=4 is used to get time of
% next hit.
%
% There can be more than one sample time providing
% they are ordered such that they are monotonically
% increasing. Only the needed sample times should be
% specified in TS. When specifying than one
% sample time, you must check for sample hits explicitly by
% seeing if
% abs(round((T-OFFSET)/PERIOD) - (T-OFFSET)/PERIOD)
% is within a specified tolerance, generally 1e-8. This
% tolerance is dependent upon your model's sampling times
% and simulation time.
%
% You can also specify that the sample time of the S-function
% is inherited from the driving block. For functions which
% change during minor steps, this is done by
% specifying SYS(7) = 1 and TS = [-1 0]. For functions which
% are held during minor steps, this is done by specifying
% SYS(7) = 1 and TS = [-1 1].
% Copyright 1990-2002 The MathWorks, Inc.
% $Revision: 1.18 $
%
% The following outlines the general structure of an S-function.
%
switch flag,
%%%%%%%%%%%%%%%%%%
% Initialization %
%%%%%%%%%%%%%%%%%%
case 0,
[sys,x0,str,ts]=mdlInitializeSizes;
%%%%%%%%%%%%%%%
% Derivatives %
%%%%%%%%%%%%%%%
case 1,
sys=mdlDerivatives(t,x,u);
%%%%%%%%%%
% Update %
%%%%%%%%%%
%case 2,
%sys=mdlUpdate(t,x,u);
%%%%%%%%%%%
% Outputs %
%%%%%%%%%%%
case 3,
sys=mdlOutputs(t,x,u);
%%%%%%%%%%%%%%%%%%%%%%%
% GetTimeOfNextVarHit %
%%%%%%%%%%%%%%%%%%%%%%%
% case 4,
%sys=mdlGetTimeOfNextVarHit(t,x,u);
%%%%%%%%%%%%%
% Terminate %
%%%%%%%%%%%%%
% case 9,
% sys=mdlTerminate(t,x,u);
case { 2, 4, 9 }
sys = []; % Unused flags
%%%%%%%%%%%%%%%%%%%%
% Unexpected flags %
%%%%%%%%%%%%%%%%%%%%
otherwise
error(['Unhandled flag = ',num2str(flag)]);
end
% end sfuntmpl
%
%=============================================================================
% mdlInitializeSizes
% Return the sizes, initial conditions, and sample times for the S-function.
%=============================================================================
%
function [sys,x0,str,ts]=mdlInitializeSizes
global etavoigt1;
etavoigt1=[5; 5; 5; 5];
global muivoigt1;
%muivoigt1=1; %% Environment Series Spring 1%%
muivoigt1=10; %% Environment Series Spring 2%%
%muivoigt1=20; %% Environment Series Spring 3%%
%muivoigt1=30; %% Environment Series Spring 4%%
%muivoigt1=40; %% Environment Series Spring 5%%
%muivoigt1=50; %% Environment Series Spring 6%%
global muovoigt1;
muovoigt1=1; %% Environment Parallel Spring 1%%
%muovoigt1=10; %% Environment Parallel Spring 2%%
%muovoigt1=20; %% Environment Parallel Spring 3%%
%muovoigt1=30; %% Environment Parallel Spring 4%%
%muovoigt1=40; %% Environment Parallel Spring 5%%
%muovoigt1=50; %% Environment Parallel Spring 6%%
global j1;
j1=0;
global A1;
global B1;
global C1;
global D1;
%
% call simsizes for a sizes structure, fill it in and convert it to a
% sizes array.
%
% Note that in this example, the values are hard coded. This is not a
% recommended practice as the characteristics of the block are typically
% defined by the S-function parameters.
%
sizes = simsizes;
sizes.NumContStates = 1;
sizes.NumDiscStates = 0;
sizes.NumOutputs = 1;
sizes.NumInputs = 1;
sizes.DirFeedthrough = 1;
sizes.NumSampleTimes = 1; % at least one sample time is needed
sys = simsizes(sizes);
%
% initialize the initial conditions
%
x0 = 0;
%
% str is always an empty matrix
%
str = [];
%
% initialize the array of sample times
%
ts = [0 0];
% end mdlInitializeSizes
%
%=============================================================================
% mdlDerivatives
% Return the derivatives for the continuous states.
%=============================================================================
%
function sys=mdlDerivatives(t,x,u)
global etavoigt1;
global muivoigt1;
global muovoigt1;
global j1;
global A1;
global B1;
global C1;
global D1;
j1=j1+1;
if (j1<=4)
A1 = -muivoigt1/etavoigt1(j1);
B1 = muivoigt1/etavoigt1(j1);
elseif (j>4)
A1 = -muivoigt1/etavoigt1(4);
B1 = muivoigt1/etavoigt1(4);
end
sys = A1*x + B1*u;
% end mdlDerivatives
%
%=============================================================================
% mdlUpdate
% Handle discrete state updates, sample time hits, and major time step
% requirements.
%=============================================================================
%
%function sys=mdlUpdate(t,x,u)
% end mdlUpdate
%
%=============================================================================
% mdlOutputs
% Return the block outputs.
%=============================================================================
%
function sys=mdlOutputs(t,x,u)
global etavoigt1;
global muivoigt1;
global muovoigt1;
global j1;
global A1;
global B1;
global C1;
global D1;
C1 = 1;
D1 = 0;
sys = C1*x + D1*u;
%end mdlOutputs
%
%=============================================================================
% mdlGetTimeOfNextVarHit
% Return the time of the next hit for this block. Note that the result is
% absolute time. Note that this function is only used when you specify a
% variable discrete-time sample time [-2 0] in the sample time array in
% mdlInitializeSizes.
%=============================================================================
%
%function sys=mdlGetTimeOfNextVarHit(t,x,u)
%sampleTime = 1; % Example, set the next hit to be one second later.
%sys = t + sampleTime;
% end mdlGetTimeOfNextVarHit
%
%=============================================================================
% mdlTerminate
% Perform any end of simulation tasks.
%=============================================================================
%
%function sys=mdlTerminate(t,x,u)
%sys = [];
% end mdlTerminate
| {
"content_hash": "19fe09b58982522e52b79dd8c5a29236",
"timestamp": "",
"source": "github",
"line_count": 317,
"max_line_length": 78,
"avg_line_length": 29.321766561514195,
"alnum_prop": 0.5383539537385691,
"repo_name": "tapomayukh/projects_in_matlab",
"id": "69bce0b35361ca01a8180ca9986ce4a242e8d66c",
"size": "9295",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "sandbox_tapo/Master's Thesis/Passivity Analysis/FOR ICAR/Passivity Analysis test3/var_eta1_tc.m",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "CSS",
"bytes": "45324"
},
{
"name": "HTML",
"bytes": "33011"
},
{
"name": "M",
"bytes": "64117"
},
{
"name": "Mathematica",
"bytes": "291634"
},
{
"name": "Matlab",
"bytes": "2677491"
},
{
"name": "Objective-C",
"bytes": "285"
}
],
"symlink_target": ""
} |
import unittest
from c7n.resources import ec2
from c7n.resources.ec2 import actions, QueryFilter
from c7n import tags, utils
from .common import BaseTest
class TestTagAugmentation(BaseTest):
def test_tag_augment_empty(self):
session_factory = self.replay_flight_data(
'test_ec2_augment_tag_empty')
# recording was modified to be sans tags
ec2 = session_factory().client('ec2')
policy = self.load_policy({
'name': 'ec2-tags',
'resource': 'ec2'},
session_factory=session_factory)
resources = policy.run()
self.assertEqual(len(resources), 0)
def test_tag_augment(self):
session_factory = self.replay_flight_data(
'test_ec2_augment_tags')
# recording was modified to be sans tags
ec2 = session_factory().client('ec2')
policy = self.load_policy({
'name': 'ec2-tags',
'resource': 'ec2',
'filters': [
{'tag:Env': 'Production'}]},
session_factory=session_factory)
resources = policy.run()
self.assertEqual(len(resources), 1)
class TestMetricFilter(BaseTest):
def test_metric_filter(self):
session_factory = self.replay_flight_data(
'test_ec2_metric')
ec2 = session_factory().client('ec2')
policy = self.load_policy({
'name': 'ec2-utilization',
'resource': 'ec2',
'filters': [
{'type': 'metrics',
'name': 'CPUUtilization',
'days': 3,
'value': 1.5}
]},
session_factory=session_factory)
resources = policy.run()
self.assertEqual(len(resources), 1)
class TestTagTrim(BaseTest):
def test_ec2_tag_trim(self):
self.patch(tags.TagTrim, 'max_tag_count', 10)
session_factory = self.replay_flight_data(
'test_ec2_tag_trim')
ec2 = session_factory().client('ec2')
start_tags = {
t['Key']: t['Value'] for t in
ec2.describe_tags(
Filters=[{'Name': 'resource-id',
'Values': ['i-fdb01920']}])['Tags']}
policy = self.load_policy({
'name': 'ec2-tag-trim',
'resource': 'ec2',
'filters': [
{'type': 'tag-count', 'count': 10}],
'actions': [
{'type': 'tag-trim',
'space': 1,
'preserve': [
'Name',
'Env',
'Account',
'Platform',
'Classification',
'Planet'
]}
]},
session_factory=session_factory)
resources = policy.run()
self.assertEqual(len(resources), 1)
end_tags = {
t['Key']: t['Value'] for t in
ec2.describe_tags(
Filters=[{'Name': 'resource-id',
'Values': ['i-fdb01920']}])['Tags']}
self.assertEqual(len(start_tags)-1, len(end_tags))
self.assertTrue('Containers' in start_tags)
self.assertFalse('Containers' in end_tags)
class TestVolumeFilter(BaseTest):
def test_ec2_attached_ebs_filter(self):
session_factory = self.replay_flight_data(
'test_ec2_attached_ebs_filter')
policy = self.load_policy({
'name': 'ec2-unencrypted-vol',
'resource': 'ec2',
'filters': [
{'type': 'ebs',
'key': 'Encrypted',
'value': False}]},
session_factory=session_factory)
resources = policy.run()
self.assertEqual(len(resources), 1)
# DISABLED / Re-record flight data on public account
def test_ec2_attached_volume_skip_block(self):
session_factory = self.replay_flight_data(
'test_ec2_attached_ebs_filter')
policy = self.load_policy({
'name': 'ec2-unencrypted-vol',
'resource': 'ec2',
'filters': [
{'type': 'ebs',
'skip-devices': ['/dev/sda1', '/dev/xvda', '/dev/sdb1'],
'key': 'Encrypted',
'value': False}]},
session_factory=session_factory)
resources = policy.run()
self.assertEqual(len(resources), 0)
class TestResizeInstance(BaseTest):
def test_ec2_resize(self):
# preconditions - three instances (2 m4.4xlarge, 1 m4.1xlarge)
# one of the instances stopped
session_factory = self.replay_flight_data('test_ec2_resize')
policy = self.load_policy({
'name': 'ec2-resize',
'resource': 'ec2',
'filters': [
{'type': 'value',
'key': 'State.Name',
'value': ['running', 'stopped'],
'op': 'in'},
{'type': 'value',
'key': 'InstanceType',
'value': ['m4.2xlarge', 'm4.4xlarge'],
'op': 'in'},
],
'actions': [
{'type': 'resize',
'restart': True,
'default': 'm4.large',
'type-map': {
'm4.4xlarge': 'm4.2xlarge'}}]
}, session_factory=session_factory)
resources = policy.run()
self.assertEqual(len(resources), 3)
stopped, running = [], []
for i in resources:
if i['State']['Name'] == 'running':
running.append(i['InstanceId'])
if i['State']['Name'] == 'stopped':
stopped.append(i['InstanceId'])
instances = utils.query_instances(
session_factory(),
InstanceIds=[r['InstanceId'] for r in resources])
cur_stopped, cur_running = [], []
for i in instances:
if i['State']['Name'] == 'running':
cur_running.append(i['InstanceId'])
if i['State']['Name'] == 'stopped':
cur_stopped.append(i['InstanceId'])
cur_running.sort()
running.sort()
self.assertEqual(cur_stopped, stopped)
self.assertEqual(cur_running, running)
instance_types = [i['InstanceType'] for i in instances]
instance_types.sort()
self.assertEqual(
instance_types,
list(sorted(['m4.large', 'm4.2xlarge', 'm4.2xlarge'])))
class TestStateTransitionAgeFilter(BaseTest):
def test_ec2_state_transition_age(self):
session_factory = self.replay_flight_data(
'test_ec2_state_transition_age_filter'
)
policy = self.load_policy({
'name': 'ec2-state-transition-age',
'resource': 'ec2',
'filters': [
{'State.Name': 'running'},
{'type': 'state-age',
'days': 30}]},
session_factory=session_factory)
resources = policy.run()
#compare stateTransition reason to expected
self.assertEqual(len(resources), 1)
self.assertEqual(resources[0]['StateTransitionReason'], 'User initiated (2015-11-25 10:11:55 GMT)')
class TestImageAgeFilter(BaseTest):
def test_ec2_image_age(self):
session_factory = self.replay_flight_data(
'test_ec2_image_age_filter')
policy = self.load_policy({
'name': 'ec2-image-age',
'resource': 'ec2',
'filters': [
{'State.Name': 'running'},
{'type': 'image-age',
'days': 30}]},
session_factory=session_factory)
resources = policy.run()
self.assertEqual(len(resources), 1)
class TestImageFilter(BaseTest):
def test_ec2_image(self):
session_factory = self.replay_flight_data(
'test_ec2_image_filter')
policy = self.load_policy({
'name': 'ec2-image',
'resource': 'ec2',
'filters': [
{'type': 'image', 'key': 'Public', 'value': True}
]},
session_factory=session_factory)
resources = policy.run()
self.assertEqual(len(resources), 1)
self.assertEqual(resources[0]['InstanceId'], 'i-039628786cabe8c16')
class TestInstanceAge(BaseTest):
# placebo doesn't record tz information
def test_ec2_instance_age(self):
session_factory = self.replay_flight_data(
'test_ec2_instance_age_filter')
policy = self.load_policy({
'name': 'ec2-instance-age',
'resource': 'ec2',
'filters': [
{'State.Name': 'running'},
{'type': 'instance-age',
'days': 0}]},
session_factory=session_factory)
resources = policy.run()
self.assertEqual(len(resources), 1)
class TestTag(BaseTest):
def test_ec2_tag(self):
session_factory = self.replay_flight_data(
'test_ec2_mark')
policy = self.load_policy({
'name': 'ec2-test-mark',
'resource': 'ec2',
'filters': [
{'State.Name': 'running'}],
'actions': [
{'type': 'tag',
'key': 'Testing',
'value': 'Testing123'}]},
session_factory=session_factory)
resources = policy.run()
self.assertEqual(len(resources), 1)
def test_ec2_untag(self):
session_factory = self.replay_flight_data(
'test_ec2_untag')
policy = self.load_policy({
'name': 'ec2-test-unmark',
'resource': 'ec2',
'filters': [
{'tag:Testing': 'not-null'}],
'actions': [
{'type': 'remove-tag',
'key': 'Testing'}]},
session_factory=session_factory)
resources = policy.run()
self.assertEqual(len(resources), 1)
class TestStop(BaseTest):
def test_ec2_stop(self):
session_factory = self.replay_flight_data(
'test_ec2_stop')
policy = self.load_policy({
'name': 'ec2-test-stop',
'resource': 'ec2',
'filters': [
{'tag:Testing': 'not-null'}],
'actions': [
{'type': 'stop'}]},
session_factory=session_factory)
resources = policy.run()
self.assertEqual(len(resources), 1)
class TestStart(BaseTest):
def test_ec2_start(self):
session_factory = self.replay_flight_data(
'test_ec2_start')
policy = self.load_policy({
'name': 'ec2-test-start',
'resource': 'ec2',
'filters': [],
'actions': [
{'type': 'start'}]},
session_factory=session_factory)
resources = policy.run()
self.assertEqual(len(resources), 2)
class TestOr(BaseTest):
def test_ec2_or_condition(self):
session_factory = self.replay_flight_data(
'test_ec2_stop')
policy = self.load_policy({
'name': 'ec2-test-snapshot',
'resource': 'ec2',
'filters': [
{"or": [
{"tag:Name": "CompileLambda"},
{"tag:Name": "Spinnaker"}]}]
}, session_factory=session_factory)
resources = policy.run()
self.assertEqual(len(resources), 2)
self.assertEqual(
sorted([r['InstanceId'] for r in resources]),
[u'i-13413bd7', u'i-1aebf7c0'])
class TestSnapshot(BaseTest):
def test_ec2_snapshot_no_copy_tags(self):
session_factory = self.replay_flight_data(
'test_ec2_snapshot')
policy = self.load_policy({
'name': 'ec2-test-snapshot',
'resource': 'ec2',
'filters': [
{'tag:Name': 'CompileLambda'}],
'actions': [
{'type': 'snapshot'}]},
session_factory=session_factory)
resources = policy.run()
self.assertEqual(len(resources), 1)
def test_ec2_snapshot_copy_tags(self):
session_factory = self.replay_flight_data(
'test_ec2_snapshot')
policy = self.load_policy({
'name': 'ec2-test-snapshot',
'resource': 'ec2',
'filters': [
{'tag:Name': 'CompileLambda'}],
'actions': [
{'type': 'snapshot', 'copy-tags': ['ASV' 'Testing123']}]},
session_factory=session_factory)
resources = policy.run()
self.assertEqual(len(resources), 1)
class TestEC2QueryFilter(unittest.TestCase):
def test_parse(self):
self.assertEqual(QueryFilter.parse([]), [])
x = QueryFilter.parse(
[{'instance-state-name': 'running'}])
self.assertEqual(
x[0].query(),
{'Name': 'instance-state-name', 'Values': ['running']})
self.assertTrue(
isinstance(
QueryFilter.parse(
[{'tag:ASV': 'REALTIMEMSG'}])[0],
QueryFilter))
self.assertRaises(
ValueError,
QueryFilter.parse,
[{'tag:ASV': None}])
class TestTerminate(BaseTest):
def test_ec2_terminate(self):
# Test conditions: single running instance, with delete protection
session_factory = self.replay_flight_data('test_ec2_terminate')
p = self.load_policy({
'name': 'ec2-term',
'resource': 'ec2',
'filters': [{'InstanceId': 'i-017cf4e2a33b853fe'}],
'actions': [
{'type': 'terminate',
'force': True}]},
session_factory=session_factory)
resources = p.run()
self.assertEqual(len(resources), 1)
instances = utils.query_instances(
session_factory(), InstanceIds=['i-017cf4e2a33b853fe'])
self.assertEqual(instances[0]['State']['Name'], 'shutting-down')
class TestDefaultVpc(BaseTest):
def test_ec2_default_vpc(self):
session_factory = self.replay_flight_data('test_ec2_default_vpc')
p = self.load_policy(
{'name': 'ec2-default-filters',
'resource': 'ec2',
'filters': [
{'type': 'default-vpc'}]},
config={'region': 'us-west-2'},
session_factory=session_factory)
resources = p.run()
self.assertEqual(len(resources), 1)
self.assertEqual(resources[0]['InstanceId'], 'i-0bfe468063b02d018')
class TestActions(unittest.TestCase):
def test_action_construction(self):
self.assertIsInstance(
actions.factory('mark', None),
tags.Tag)
self.assertIsInstance(
actions.factory('stop', None),
ec2.Stop)
self.assertIsInstance(
actions.factory('terminate', None),
ec2.Terminate)
| {
"content_hash": "75bfe5bf155cc685046924080f0370d7",
"timestamp": "",
"source": "github",
"line_count": 459,
"max_line_length": 107,
"avg_line_length": 32.631808278867105,
"alnum_prop": 0.5102817465616237,
"repo_name": "RyanWolfe/cloud-custodian",
"id": "8d340d7345f45f510eeea10bf5d5971d0abb6a44",
"size": "15563",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "tests/test_ec2.py",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "Makefile",
"bytes": "1184"
},
{
"name": "Python",
"bytes": "845781"
}
],
"symlink_target": ""
} |
"""
AIs used to determine which move to pick in a battle.
"""
import random
class RandomMoveAi:
"""
AI which just selects any move at random from the pool of available
ones (i.e. those with PP > 0).
"""
def __init__(self, battle_creature):
self.battle_creature = battle_creature
def select_move(self):
"""
Should use struggle if the creature has no moves. Just doesn't
select a move for now.
"""
pp_moves = [move for move in self.battle_creature.creature.moves if move.pp > 0]
if len(pp_moves) == 0:
return None
return random.choice(pp_moves)
| {
"content_hash": "3c91506c48e95ad09a60c11c9809e3d4",
"timestamp": "",
"source": "github",
"line_count": 25,
"max_line_length": 88,
"avg_line_length": 26.76,
"alnum_prop": 0.5874439461883408,
"repo_name": "DaveTCode/CreatureRogue",
"id": "fea29183593ba1852ae4b67ff1ef5fa43c29b75a",
"size": "669",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "CreatureRogue/battle_ai.py",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "Python",
"bytes": "129073"
}
],
"symlink_target": ""
} |
package cognitivej.vision.face.person.action;
import cognitivej.core.RestAction;
import cognitivej.core.WorkingContext;
import cognitivej.core.error.ErrorHandler;
import cognitivej.vision.face.CognitiveContext;
import cognitivej.vision.face.person.error.PersonNotFoundErrorHandler;
import com.mashape.unirest.http.HttpMethod;
import org.apache.http.HttpStatus;
import org.jetbrains.annotations.NotNull;
import java.util.Map;
public class DeletePersonAction extends RestAction<Void> {
private final WorkingContext workingContext = new WorkingContext();
private final String personGroupId;
private final String personId;
public DeletePersonAction(@NotNull CognitiveContext cognitiveContext, @NotNull String personGroupId, @NotNull String personId) {
super(cognitiveContext);
this.personGroupId = personGroupId;
this.personId = personId;
buildContext(personGroupId);
}
private void buildContext(String id) {
workingContext.setPath("face/v1.0/persongroups/${personGroupId}/persons/${personId}")
.addPathVariable("personGroupId", id).addPathVariable("personId", personId)
.httpMethod(HttpMethod.DELETE);
}
@Override
protected WorkingContext workingContext() {
return workingContext;
}
@Override
protected void customErrorHandlers(Map<Integer, ErrorHandler> errorHandlers) {
errorHandlers.put(HttpStatus.SC_NOT_FOUND, new PersonNotFoundErrorHandler(personGroupId, personId));
}
}
| {
"content_hash": "fb35a17afbeef07d465be9030e0fd762",
"timestamp": "",
"source": "github",
"line_count": 44,
"max_line_length": 132,
"avg_line_length": 34.63636363636363,
"alnum_prop": 0.7519685039370079,
"repo_name": "CognitiveJ/cognitivej",
"id": "f000c27acae63e91f9773dcac588b3c0dfe67c0f",
"size": "13443",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "src/main/java/cognitivej/vision/face/person/action/DeletePersonAction.java",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "Groovy",
"bytes": "499204"
},
{
"name": "Java",
"bytes": "2304811"
}
],
"symlink_target": ""
} |
package xsmeral.semnet.util;
import java.io.UnsupportedEncodingException;
import java.net.MalformedURLException;
import java.net.URI;
import java.net.URISyntaxException;
import java.net.URL;
import java.net.URLDecoder;
import java.net.URLEncoder;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.SortedSet;
import java.util.TreeSet;
import java.util.logging.Level;
import java.util.logging.Logger;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import org.apache.commons.lang.StringEscapeUtils;
/**
* Provides methods for URL normalization and host equality comparison.
* @author Ron Šmeral ([email protected])
*/
public class URLUtil {
private static final String COLON = ":";
private static final String SEMICOLON = ";";
private static final String AMPERSAND = "&";
private static final String EQUALS_SIGN = "=";
private static final String PERCENT = "%";
private static final String QUESTION_MARK = "?";
private static final String FWD_SLASH = "/";
private static final String DOT = ".";
//
private static String URL_CHARSET = "UTF-8";
private static final String URLENCODED_SYMBOL_PATTERN = "%(\\p{XDigit}{2})";
private static final Pattern urlEncSymbolPattern = Pattern.compile(URLENCODED_SYMBOL_PATTERN);
// common parameters, usually of no significance to the content (session ids, analytics, etc.)
private static final String[] unwantedStrings = {"^utm_", "session"};
private static final List<Pattern> unwantedPatterns = new ArrayList<Pattern>(unwantedStrings.length);
static {
// pre-compile unwanted parameter patterns
for (String unwantedStr : unwantedStrings) {
unwantedPatterns.add(Pattern.compile(unwantedStr));
}
}
/**
* Returns scheme and authority part of URL with trailing slash.
*/
public static String fullHost(URL url) {
return "http://" + url.getAuthority() + "/";
}
public static boolean equalHosts(URL host1, URL host2, boolean full) {
return full ? host1.equals(host2) : host1.getHost().equals(host2.getHost());
}
/**
* Convenience method, calls {@link #normalize(java.net.URL) normalize}{@code (new URL(url))}.
* Tries to add {@code http://} if it's missing
* @param url String containing the url to be normalized
* @return Normalized URL, or the supplied string unchanged in case of failure
*/
public static URL normalize(String url) throws MalformedURLException {
URL normalized = null;
try {
normalized = normalize(new URL(url));
} catch (MalformedURLException ex) {
// try adding http://, may help
normalized = !url.startsWith("http://") ? normalize("http://" + url) : null;
}
return normalized;
}
/**
* Important part of every crawler - a URL normalizer. Ensures equivalence
* of different representations of the same URL.<br />
* Adheres mostly to RFC 3986 and http://dblab.ssu.ac.kr/publication/LeKi05a.pdf<br />
* Performs these steps:<br />
* <ul>
* <li>case normalization</li>
* <li>removes document fragment</li>
* <li>removes standard port number</li>
* <li>decodes unreserved characters in path</li>
* <li>parameter sorting (allows & and ; delimiters) and filtering, allows
* empty-valued and multi-valued params</li>
* <li>capitalizes percent-encoded octets</li>
* <li>normalizes path (resolves dot-segments and adds trailing slash to empty path)</li>
* <li>tries to add http:// if the input url doesn't have protocol</li>
* </ul>
*
* @author Ron Šmeral ([email protected])
*/
public static URL normalize(URL url) {
int port = url.getPort();
String path = url.getPath();
String query = sortAndCleanParams(StringEscapeUtils.unescapeHtml(url.getQuery()));
if (path == null || path.isEmpty()) {
path = FWD_SLASH;
}
try {
StringBuilder outUrlStr = new StringBuilder(url.toString().length())//
.append(url.getProtocol())//
.append("://")//
.append(url.getHost().toLowerCase())//
.append((port == 80 || port == -1) ? "" : COLON + port)//
.append(decodeUnreserved(path)).append(!query.isEmpty() ? QUESTION_MARK : "")//
.append(query);
return new URI(outUrlStr.toString()).normalize().toURL();
} catch (MalformedURLException ex) {
Logger.getLogger(URLUtil.class.getName()).log(Level.SEVERE, null, ex);
return null;
} catch (URISyntaxException ex) {
Logger.getLogger(URLUtil.class.getName()).log(Level.SEVERE, null, ex);
return null;
}
}
/**
* Used for parameter sorting and filtering
*
* @param query The query string part of URL
* @return Query string with sorted and filtered params
*/
private static String sortAndCleanParams(String query) {
if (query == null || query.isEmpty()) {
return "";
}
// determine param delimiter, can be ; or &
String delimiter = query.contains(AMPERSAND) ? AMPERSAND : query.contains(SEMICOLON) ? SEMICOLON : null;
// if there is no delimiter and the query string doesn't contain equals sign, leave the query as is
if (delimiter == null && !query.contains(EQUALS_SIGN)) {
return URLEncode(URLDecode(query));
}
// sort and filter the params
String[] params = (delimiter != null) ? query.split(delimiter) : new String[]{query};
SortedSet<Param> paramSet = new TreeSet<Param>();
for (int i = 0; i < params.length; i++) {
String paramStr = params[i];
if (!paramStr.isEmpty()) {
boolean hasEquals = paramStr.contains(EQUALS_SIGN);
int equalsPos = hasEquals ? paramStr.indexOf(EQUALS_SIGN) : -1;
int paramLen = paramStr.length();
String field = URLEncode(URLDecode(hasEquals ? paramStr.substring(0, equalsPos) : paramStr));
String value = ((hasEquals && (equalsPos != paramLen - 1)) ? URLEncode(URLDecode(paramStr.substring(equalsPos + 1, paramLen))) : "");
boolean isUnwanted = false;
for (int j = 0; j < unwantedStrings.length && !isUnwanted; j++) {
isUnwanted = isUnwanted || unwantedPatterns.get(j).matcher(field).find();
}
if (!isUnwanted) {
paramSet.add(new Param(field, value, i));
}
}
}
// put the query string back together
switch (paramSet.size()) {
case 0:
return "";
case 1:
return paramSet.first().toString();
default:
Iterator<Param> it = paramSet.iterator();
StringBuilder queryString = new StringBuilder(query.length() + 10);
queryString.append(it.next().toString());
while (it.hasNext()) {
queryString.append(delimiter).append(it.next());
}
return queryString.toString();
}
}
/**
* Replaces characters not reserved in URL, according to RFC 3986,
* which are ALPHA (%41-%5A and %61-%7A), DIGIT (%30-%39), hyphen (%2D), period (%2E),
* underscore (%5F), or tilde (%7E)
*
* @param str Input string
* @return String with percent-sequences only for reserved characters
*/
private static String decodeUnreserved(String str) {
if (str == null || str.isEmpty()) {
return "";
}
if (!str.contains(PERCENT)) {
return str;
}
Matcher m = urlEncSymbolPattern.matcher(str);
StringBuffer buf = new StringBuffer();
String rep;
while (m.find()) {
int v = Integer.parseInt(m.group(1), 16);
if ((v >= 'a' && v <= 'z') || (v >= 'A' && v <= 'Z') || (v >= '0' && v <= '9')
|| v == '-' || v == '.' || v == '_' || v == '~') {
rep = String.valueOf((char) v);
} else {
rep = m.group().toUpperCase();
}
m.appendReplacement(buf, rep);
}
m.appendTail(buf);
return buf.toString();
}
/**
* Wrapper, calls {@link URLEncoder URLEncoder}.{@link URLEncoder#encode(java.lang.String, java.lang.String) encode}{@code (str, "UTF-8")}
* @param str String to URL-encode, should be fully decoded
* @return URL-encoded string
*/
private static String URLEncode(String str) {
try {
// if the string contains at least one url-encoded symbol, it is very likely it's already url-encoded
return str.contains(PERCENT) ? str : URLEncoder.encode(str, URL_CHARSET);
} catch (UnsupportedEncodingException ex) {
Logger.getLogger(URLUtil.class.getName()).log(Level.SEVERE, null, ex);
return str;
}
}
/**
* Wrapper, calls {@link URLDecoder URLDecoder}.{@link URLDecoder#decode(java.lang.String, java.lang.String) decode}{@code (str, "UTF-8")}
* @param str String to URL-decode
* @return URL-decoded string
*/
private static String URLDecode(String str) {
try {
return URLDecoder.decode(str, URL_CHARSET);
} catch (UnsupportedEncodingException ex) {
Logger.getLogger(URLUtil.class.getName()).log(Level.SEVERE, null, ex);
return str;
}
}
/**
* Represents one query string param. Implements Comparable,
* the sorting keys are field, value and original position in query string
*/
private static final class Param implements Comparable {
private int origPosition;
private String field;
private String value;
public Param() {
}
public Param(String field, String value, int origPosition) {
this.field = field;
this.value = value;
}
public Param(String field, String value) {
this(field, value, 0);
}
public int getOrigPosition() {
return origPosition;
}
public void setOrigPosition(int origPosition) {
this.origPosition = origPosition;
}
public String getField() {
return field;
}
public void setField(final String field) {
this.field = field;
}
public String getValue() {
return value;
}
public void setValue(final String value) {
this.value = value;
}
/**
* Compares by field, value and original position in query string
* @param obj Other object
* @return Result of comparison
*/
@Override
public int compareTo(Object obj) {
Param o = (Param) obj;
int fieldDiff = this.field.compareTo(o.getField());
if (fieldDiff != 0) {
return fieldDiff;
} else {
int valDiff = this.value.compareTo(o.getValue());
if (valDiff != 0) {
return valDiff;
} else {
return this.origPosition - o.getOrigPosition();
}
}
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (getClass() != obj.getClass()) {
return false;
}
final Param other = (Param) obj;
if (this.origPosition != other.origPosition) {
return false;
}
if ((this.field == null) ? (other.field != null) : !this.field.equals(other.field)) {
return false;
}
if ((this.value == null) ? (other.value != null) : !this.value.equals(other.value)) {
return false;
}
return true;
}
@Override
public int hashCode() {
int hash = 3;
hash = 59 * hash + this.origPosition;
hash = 59 * hash + (this.field != null ? this.field.hashCode() : 0);
hash = 59 * hash + (this.value != null ? this.value.hashCode() : 0);
return hash;
}
@Override
public String toString() {
return new StringBuilder(field.length() + value.length() + 1).append(this.field).append(EQUALS_SIGN).append(this.value).toString();
}
}
}
| {
"content_hash": "3075c66c80c816c15b1910f98b3b1bfa",
"timestamp": "",
"source": "github",
"line_count": 344,
"max_line_length": 149,
"avg_line_length": 37.23837209302326,
"alnum_prop": 0.5683060109289617,
"repo_name": "rsmeral/semnet",
"id": "072c989d3bf214eea2c0fc34dcc13747ca0d3f37",
"size": "12812",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "SemNet/src/xsmeral/semnet/util/URLUtil.java",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "Java",
"bytes": "330616"
}
],
"symlink_target": ""
} |
package org.javabits.yar;
import java.util.EventListener;
/**
* TODO comment
* Date: 5/29/13
* Time: 10:31 PM
*
* @author Romain Gilles
*/
public interface TypeListener extends EventListener {
void typeChanged(TypeEvent typeEvent);
}
| {
"content_hash": "059d0d6a2b53e9709768d2312aa0bcb4",
"timestamp": "",
"source": "github",
"line_count": 14,
"max_line_length": 53,
"avg_line_length": 17.571428571428573,
"alnum_prop": 0.7154471544715447,
"repo_name": "javabits/yar",
"id": "e3bab14fedb0a8a21c03ac3fa2a6de9615c16564",
"size": "246",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "yar-api/src/main/java/org/javabits/yar/TypeListener.java",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "Java",
"bytes": "400825"
}
],
"symlink_target": ""
} |
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en" lang="en-us">
{% include head.html %}
<body class=theme-base-0d>
{% include sidebar.html %}
<!-- Wrap is the content to shift when toggling the sidebar. We wrap the
content to avoid any CSS collisions with our real content. -->
<div class="wrap">
<div class="masthead">
<div class="container">
<label for="sidebar-checkbox" class="sidebar-toggle"></label>
<h3 class="masthead-title">
<a href="{{ site.baseurl }}" title="Home">{{ site.title }}</a>
<small>{{ site.tagline }}</small>
</h3>
</div>
</div>
<div class="container content">
{{ content }}
</div>
</div>
</body>
</html>
| {
"content_hash": "1b16734d043d2190df58f2b4e27ef8e5",
"timestamp": "",
"source": "github",
"line_count": 30,
"max_line_length": 76,
"avg_line_length": 26.333333333333332,
"alnum_prop": 0.5481012658227848,
"repo_name": "sunghlin/blog",
"id": "486cb71780eeba8a960907c717b9fcee6f5602f3",
"size": "790",
"binary": false,
"copies": "1",
"ref": "refs/heads/gh-pages",
"path": "_layouts/default.html",
"mode": "33261",
"license": "mit",
"language": [
{
"name": "CSS",
"bytes": "20649"
}
],
"symlink_target": ""
} |
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Interfaces;
namespace Html
{
public class HTMLElementFactory : IElementFactory
{
public IElement CreateElement(string name)
{
throw new NotImplementedException();
}
public IElement CreateElement(string name, string content)
{
throw new NotImplementedException();
}
public ITable CreateTable(int rows, int cols)
{
throw new NotImplementedException();
}
}
}
| {
"content_hash": "af3e3eb4b52c1f93964b8fdc0a75f073",
"timestamp": "",
"source": "github",
"line_count": 27,
"max_line_length": 66,
"avg_line_length": 22.296296296296298,
"alnum_prop": 0.6378737541528239,
"repo_name": "NikolaiMishev/Telerik-Academy",
"id": "aabf98074948c4f6fe822bd271ffd6d9d141f42f",
"size": "604",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "Module-1/03.CSharp OOP/Html/Html/HTMLElementFactory.cs",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "C#",
"bytes": "3091339"
},
{
"name": "CSS",
"bytes": "35217"
},
{
"name": "HTML",
"bytes": "98505"
},
{
"name": "JavaScript",
"bytes": "257776"
}
],
"symlink_target": ""
} |
<?php
declare(strict_types=1);
namespace Phan\Output\Filter;
use Phan\IssueInstance;
use Phan\Output\IssueFilterInterface;
/**
* This is a filter which permits any IssueInstance to be output.
*/
final class AnyFilter implements IssueFilterInterface
{
/**
* @param IssueInstance $issue (@phan-unused-param)
*/
public function supports(IssueInstance $issue): bool
{
return true;
}
}
| {
"content_hash": "01a60dd6a20252d21c8e672e1e86dff1",
"timestamp": "",
"source": "github",
"line_count": 23,
"max_line_length": 65,
"avg_line_length": 18.347826086956523,
"alnum_prop": 0.6919431279620853,
"repo_name": "etsy/phan",
"id": "346011887b45224867182e82f6ece94f31e1080f",
"size": "422",
"binary": false,
"copies": "2",
"ref": "refs/heads/master",
"path": "src/Phan/Output/Filter/AnyFilter.php",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "PHP",
"bytes": "2492602"
},
{
"name": "Shell",
"bytes": "5117"
},
{
"name": "Vim script",
"bytes": "851"
}
],
"symlink_target": ""
} |
<?php
namespace Carbon\Traits;
/**
* Trait Week.
*
* week and ISO week number, year and count in year.
*
* Depends on the following properties:
*
* @property int $daysInYear
* @property int $dayOfWeek
* @property int $dayOfYear
* @property int $year
*
* Depends on the following methods:
*
* @method static addWeeks(int $weeks = 1)
* @method static copy()
* @method static dayOfYear(int $dayOfYear)
* @method string getTranslationMessage(string $key, ?string $locale = null, ?string $default = null, $translator = null)
* @method static next(int|string $day = null)
* @method static startOfWeek(int $day = 1)
* @method static subWeeks(int $weeks = 1)
* @method static year(int $year = null)
*/
trait Week
{
/**
* Set/get the week number of year using given first day of week and first
* day of year included in the first week. Or use ISO format if no settings
* given.
*
* @param int|null $year if null, act as a getter, if not null, set the year and return current instance.
* @param int|null $dayOfWeek first date of week from 0 (Sunday) to 6 (Saturday)
* @param int|null $dayOfYear first day of year included in the week #1
*
* @return int|static
*/
public function isoWeekYear($year = null, $dayOfWeek = null, $dayOfYear = null)
{
return $this->weekYear(
$year,
$dayOfWeek ?? 1,
$dayOfYear ?? 4
);
}
/**
* Set/get the week number of year using given first day of week and first
* day of year included in the first week. Or use US format if no settings
* given (Sunday / Jan 6).
*
* @param int|null $year if null, act as a getter, if not null, set the year and return current instance.
* @param int|null $dayOfWeek first date of week from 0 (Sunday) to 6 (Saturday)
* @param int|null $dayOfYear first day of year included in the week #1
*
* @return int|static
*/
public function weekYear($year = null, $dayOfWeek = null, $dayOfYear = null)
{
$dayOfWeek = $dayOfWeek ?? $this->getTranslationMessage('first_day_of_week') ?? 0;
$dayOfYear = $dayOfYear ?? $this->getTranslationMessage('day_of_first_week_of_year') ?? 1;
if ($year !== null) {
$year = (int) round($year);
if ($this->weekYear(null, $dayOfWeek, $dayOfYear) === $year) {
return $this->avoidMutation();
}
$week = $this->week(null, $dayOfWeek, $dayOfYear);
$day = $this->dayOfWeek;
$date = $this->year($year);
switch ($date->weekYear(null, $dayOfWeek, $dayOfYear) - $year) {
case 1:
$date = $date->subWeeks(26);
break;
case -1:
$date = $date->addWeeks(26);
break;
}
$date = $date->addWeeks($week - $date->week(null, $dayOfWeek, $dayOfYear))->startOfWeek($dayOfWeek);
if ($date->dayOfWeek === $day) {
return $date;
}
return $date->next($day);
}
$year = $this->year;
$day = $this->dayOfYear;
$date = $this->avoidMutation()->dayOfYear($dayOfYear)->startOfWeek($dayOfWeek);
if ($date->year === $year && $day < $date->dayOfYear) {
return $year - 1;
}
$date = $this->avoidMutation()->addYear()->dayOfYear($dayOfYear)->startOfWeek($dayOfWeek);
if ($date->year === $year && $day >= $date->dayOfYear) {
return $year + 1;
}
return $year;
}
/**
* Get the number of weeks of the current week-year using given first day of week and first
* day of year included in the first week. Or use ISO format if no settings
* given.
*
* @param int|null $dayOfWeek first date of week from 0 (Sunday) to 6 (Saturday)
* @param int|null $dayOfYear first day of year included in the week #1
*
* @return int
*/
public function isoWeeksInYear($dayOfWeek = null, $dayOfYear = null)
{
return $this->weeksInYear(
$dayOfWeek ?? 1,
$dayOfYear ?? 4
);
}
/**
* Get the number of weeks of the current week-year using given first day of week and first
* day of year included in the first week. Or use US format if no settings
* given (Sunday / Jan 6).
*
* @param int|null $dayOfWeek first date of week from 0 (Sunday) to 6 (Saturday)
* @param int|null $dayOfYear first day of year included in the week #1
*
* @return int
*/
public function weeksInYear($dayOfWeek = null, $dayOfYear = null)
{
$dayOfWeek = $dayOfWeek ?? $this->getTranslationMessage('first_day_of_week') ?? 0;
$dayOfYear = $dayOfYear ?? $this->getTranslationMessage('day_of_first_week_of_year') ?? 1;
$year = $this->year;
$start = $this->avoidMutation()->dayOfYear($dayOfYear)->startOfWeek($dayOfWeek);
$startDay = $start->dayOfYear;
if ($start->year !== $year) {
$startDay -= $start->daysInYear;
}
$end = $this->avoidMutation()->addYear()->dayOfYear($dayOfYear)->startOfWeek($dayOfWeek);
$endDay = $end->dayOfYear;
if ($end->year !== $year) {
$endDay += $this->daysInYear;
}
return (int) round(($endDay - $startDay) / 7);
}
/**
* Get/set the week number using given first day of week and first
* day of year included in the first week. Or use US format if no settings
* given (Sunday / Jan 6).
*
* @param int|null $week
* @param int|null $dayOfWeek
* @param int|null $dayOfYear
*
* @return int|static
*/
public function week($week = null, $dayOfWeek = null, $dayOfYear = null)
{
$date = $this;
$dayOfWeek = $dayOfWeek ?? $this->getTranslationMessage('first_day_of_week') ?? 0;
$dayOfYear = $dayOfYear ?? $this->getTranslationMessage('day_of_first_week_of_year') ?? 1;
if ($week !== null) {
return $date->addWeeks(round($week) - $this->week(null, $dayOfWeek, $dayOfYear));
}
$start = $date->avoidMutation()->dayOfYear($dayOfYear)->startOfWeek($dayOfWeek);
$end = $date->avoidMutation()->startOfWeek($dayOfWeek);
if ($start > $end) {
$start = $start->subWeeks(26)->dayOfYear($dayOfYear)->startOfWeek($dayOfWeek);
}
$week = (int) ($start->diffInDays($end) / 7 + 1);
return $week > $end->weeksInYear($dayOfWeek, $dayOfYear) ? 1 : $week;
}
/**
* Get/set the week number using given first day of week and first
* day of year included in the first week. Or use ISO format if no settings
* given.
*
* @param int|null $week
* @param int|null $dayOfWeek
* @param int|null $dayOfYear
*
* @return int|static
*/
public function isoWeek($week = null, $dayOfWeek = null, $dayOfYear = null)
{
return $this->week(
$week,
$dayOfWeek ?? 1,
$dayOfYear ?? 4
);
}
}
| {
"content_hash": "a6220f967e23690a4e5a720671029368",
"timestamp": "",
"source": "github",
"line_count": 212,
"max_line_length": 121,
"avg_line_length": 33.79716981132076,
"alnum_prop": 0.5683182135380321,
"repo_name": "briannesbitt/Carbon",
"id": "6f1481456aca11bb99000ba172f10b816d9de15b",
"size": "7389",
"binary": false,
"copies": "22",
"ref": "refs/heads/master",
"path": "src/Carbon/Traits/Week.php",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "Batchfile",
"bytes": "93"
},
{
"name": "PHP",
"bytes": "13841845"
}
],
"symlink_target": ""
} |
package com.example.taxihelper.widget;
import android.content.Context;
import android.content.res.TypedArray;
import android.graphics.Bitmap;
import android.graphics.BitmapShader;
import android.graphics.Canvas;
import android.graphics.Color;
import android.graphics.Matrix;
import android.graphics.Paint;
import android.graphics.RectF;
import android.graphics.Shader;
import android.graphics.drawable.BitmapDrawable;
import android.graphics.drawable.ColorDrawable;
import android.graphics.drawable.Drawable;
import android.net.Uri;
import android.util.AttributeSet;
import android.widget.ImageView;
import com.example.taxihelper.R;
public class CircleImageView extends ImageView {
private static final ScaleType SCALE_TYPE = ScaleType.CENTER_CROP;
private static final Bitmap.Config BITMAP_CONFIG = Bitmap.Config.ARGB_8888;
private static final int COLORDRAWABLE_DIMENSION = 2;
private static final int DEFAULT_BORDER_WIDTH = 0;
private static final int DEFAULT_BORDER_COLOR = Color.BLACK;
private final RectF mDrawableRect = new RectF();
private final RectF mBorderRect = new RectF();
private final Matrix mShaderMatrix = new Matrix();
private final Paint mBitmapPaint = new Paint();
private final Paint mBorderPaint = new Paint();
private int mBorderColor = DEFAULT_BORDER_COLOR;
private int mBorderWidth = DEFAULT_BORDER_WIDTH;
private Bitmap mBitmap;
private BitmapShader mBitmapShader;
private int mBitmapWidth;
private int mBitmapHeight;
private float mDrawableRadius;
private float mBorderRadius;
private boolean mReady;
private boolean mSetupPending;
public CircleImageView(Context context) {
super(context);
init();
}
public CircleImageView(Context context, AttributeSet attrs) {
this(context, attrs, 0);
}
public CircleImageView(Context context, AttributeSet attrs, int defStyle) {
super(context, attrs, defStyle);
TypedArray a = context.obtainStyledAttributes(attrs, R.styleable.CircleImageView, defStyle, 0);
mBorderWidth = a.getDimensionPixelSize(R.styleable.CircleImageView_border_width, DEFAULT_BORDER_WIDTH);
mBorderColor = a.getColor(R.styleable.CircleImageView_border_color, DEFAULT_BORDER_COLOR);
a.recycle();
init();
}
private void init() {
super.setScaleType(SCALE_TYPE);
mReady = true;
if (mSetupPending) {
setup();
mSetupPending = false;
}
}
@Override
public ScaleType getScaleType() {
return SCALE_TYPE;
}
@Override
public void setScaleType(ScaleType scaleType) {
if (scaleType != SCALE_TYPE) {
throw new IllegalArgumentException(String.format("ScaleType %s not supported.", scaleType));
}
}
@Override
public void setAdjustViewBounds(boolean adjustViewBounds) {
if (adjustViewBounds) {
throw new IllegalArgumentException("adjustViewBounds not supported.");
}
}
@Override
protected void onDraw(Canvas canvas) {
if (getDrawable() == null) {
return;
}
canvas.drawCircle(getWidth() / 2, getHeight() / 2, mDrawableRadius, mBitmapPaint);
if (mBorderWidth != 0) {
canvas.drawCircle(getWidth() / 2, getHeight() / 2, mBorderRadius, mBorderPaint);
}
}
@Override
protected void onSizeChanged(int w, int h, int oldw, int oldh) {
super.onSizeChanged(w, h, oldw, oldh);
setup();
}
public int getBorderColor() {
return mBorderColor;
}
public void setBorderColor(int borderColor) {
if (borderColor == mBorderColor) {
return;
}
mBorderColor = borderColor;
mBorderPaint.setColor(mBorderColor);
invalidate();
}
public int getBorderWidth() {
return mBorderWidth;
}
public void setBorderWidth(int borderWidth) {
if (borderWidth == mBorderWidth) {
return;
}
mBorderWidth = borderWidth;
setup();
}
@Override
public void setImageBitmap(Bitmap bm) {
super.setImageBitmap(bm);
mBitmap = bm;
setup();
}
@Override
public void setImageDrawable(Drawable drawable) {
super.setImageDrawable(drawable);
mBitmap = getBitmapFromDrawable(drawable);
setup();
}
@Override
public void setImageResource(int resId) {
super.setImageResource(resId);
mBitmap = getBitmapFromDrawable(getDrawable());
setup();
}
@Override
public void setImageURI(Uri uri) {
super.setImageURI(uri);
mBitmap = getBitmapFromDrawable(getDrawable());
setup();
}
private Bitmap getBitmapFromDrawable(Drawable drawable) {
if (drawable == null) {
return null;
}
if (drawable instanceof BitmapDrawable) {
return ((BitmapDrawable) drawable).getBitmap();
}
try {
Bitmap bitmap;
if (drawable instanceof ColorDrawable) {
bitmap = Bitmap.createBitmap(COLORDRAWABLE_DIMENSION, COLORDRAWABLE_DIMENSION, BITMAP_CONFIG);
} else {
bitmap = Bitmap.createBitmap(drawable.getIntrinsicWidth(), drawable.getIntrinsicHeight(), BITMAP_CONFIG);
}
Canvas canvas = new Canvas(bitmap);
drawable.setBounds(0, 0, canvas.getWidth(), canvas.getHeight());
drawable.draw(canvas);
return bitmap;
} catch (OutOfMemoryError e) {
return null;
}
}
private void setup() {
if (!mReady) {
mSetupPending = true;
return;
}
if (mBitmap == null) {
return;
}
mBitmapShader = new BitmapShader(mBitmap, Shader.TileMode.CLAMP, Shader.TileMode.CLAMP);
mBitmapPaint.setAntiAlias(true);
mBitmapPaint.setShader(mBitmapShader);
mBorderPaint.setStyle(Paint.Style.STROKE);
mBorderPaint.setAntiAlias(true);
mBorderPaint.setColor(mBorderColor);
mBorderPaint.setStrokeWidth(mBorderWidth);
mBitmapHeight = mBitmap.getHeight();
mBitmapWidth = mBitmap.getWidth();
mBorderRect.set(0, 0, getWidth(), getHeight());
mBorderRadius = Math.min((mBorderRect.height() - mBorderWidth) / 2, (mBorderRect.width() - mBorderWidth) / 2);
mDrawableRect.set(mBorderWidth, mBorderWidth, mBorderRect.width() - mBorderWidth, mBorderRect.height() - mBorderWidth);
mDrawableRadius = Math.min(mDrawableRect.height() / 2, mDrawableRect.width() / 2);
updateShaderMatrix();
invalidate();
}
private void updateShaderMatrix() {
float scale;
float dx = 0;
float dy = 0;
mShaderMatrix.set(null);
if (mBitmapWidth * mDrawableRect.height() > mDrawableRect.width() * mBitmapHeight) {
scale = mDrawableRect.height() / (float) mBitmapHeight;
dx = (mDrawableRect.width() - mBitmapWidth * scale) * 0.5f;
} else {
scale = mDrawableRect.width() / (float) mBitmapWidth;
dy = (mDrawableRect.height() - mBitmapHeight * scale) * 0.5f;
}
mShaderMatrix.setScale(scale, scale);
mShaderMatrix.postTranslate((int) (dx + 0.5f) + mBorderWidth, (int) (dy + 0.5f) + mBorderWidth);
mBitmapShader.setLocalMatrix(mShaderMatrix);
}
} | {
"content_hash": "53ee02a04959c84bb0dd8f31e85fb777",
"timestamp": "",
"source": "github",
"line_count": 205,
"max_line_length": 127,
"avg_line_length": 36.4390243902439,
"alnum_prop": 0.6445783132530121,
"repo_name": "zzbb1199/TaxiHelper",
"id": "31d2943a4c2618142e58453eed3538c50de40830",
"size": "7470",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "app/src/main/java/com/example/taxihelper/widget/CircleImageView.java",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "Java",
"bytes": "384428"
}
],
"symlink_target": ""
} |
@interface SKTestClass : NSObject
@property (strong, nonatomic) NSString *testString;
- (void) printString;
@end
| {
"content_hash": "8b2f78c4ccffb0d51009fc446fb2b9b7",
"timestamp": "",
"source": "github",
"line_count": 8,
"max_line_length": 51,
"avg_line_length": 14.625,
"alnum_prop": 0.7435897435897436,
"repo_name": "subhamkhandelwal/pod-repo",
"id": "771ef78a4599bc2b83f067d35b02ef4bd46489e7",
"size": "302",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "pod-repo/SKTestClass.h",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "Objective-C",
"bytes": "1898"
},
{
"name": "Ruby",
"bytes": "1102"
}
],
"symlink_target": ""
} |
from __future__ import print_function
import argparse
import datetime
import dateutil.parser
import dateutil.tz
import gzip
import json
import logging
import os
import pickle
import sys
from collections import OrderedDict
from functools import partial
from itertools import count, groupby
from six.moves.urllib.request import urlopen, Request
logging.basicConfig(level=logging.INFO)
API_PARAMS = {
'base_url': 'https://api.github.com/repos',
'owner': 'bokeh',
'repo': 'bokeh',
}
IGNORE_ISSUE_TYPE = {
'type: discussion',
'type: tracker',
}
LOG_SECTION = OrderedDict([ # issue type label -> log section heading
('type: bug', 'bugfixes'),
('type: feature', 'features'),
('type: task', 'tasks'),
])
ISSUES_SORT_KEY = lambda issue: (issue_section_order(issue), int(issue['number']))
ISSUES_BY_SECTION = lambda issue: issue_section(issue)
#######################################
# Object Storage
#######################################
def save_object(filename, obj):
"""Compresses and pickles given object to the given filename."""
logging.info('saving {}...'.format(filename))
try:
with gzip.GzipFile(filename, 'wb') as f:
f.write(pickle.dumps(obj, 1))
except Exception as e:
logging.error('save failure: {}'.format(e))
raise
def load_object(filename):
"""Unpickles and decompresses the given filename and returns the created object."""
logging.info('loading {}...'.format(filename))
try:
with gzip.GzipFile(filename, 'rb') as f:
buf = ''
while True:
data = f.read()
if data == '':
break
buf += data
return pickle.loads(buf)
except Exception as e:
logging.error('load failure: {}'.format(e))
raise
#######################################
# Issues
#######################################
def issue_section_order(issue):
"""Returns the section order for the given issue."""
try:
return LOG_SECTION.values().index(issue_section(issue))
except:
return -1
def issue_completed(issue):
"""Returns True iff this issue is has been resolved as completed."""
labels = issue.get('labels', [])
return any(label['name'] == 'reso: completed' for label in labels)
def issue_section(issue):
"""Returns the section heading for the issue, or None if this issue should be ignored."""
labels = issue.get('labels', [])
for label in labels:
if not label['name'].startswith('type: '):
continue
if label['name'] in LOG_SECTION:
return LOG_SECTION[label['name']]
elif label['name'] in IGNORE_ISSUE_TYPE:
return None
else:
logging.warning('unknown issue type: "{}" for: {}'.format(label['name'], issue_line(issue)))
return None
def issue_tags(issue):
"""Returns list of tags for this issue."""
labels = issue.get('labels', [])
return [label['name'].replace('tag: ', '') for label in labels if label['name'].startswith('tag: ')]
def closed_issue(issue, after=None):
"""Returns True iff this issue was closed after given date. If after not given, only checks if issue is closed."""
if issue['state'] == 'closed':
if after is None or parse_timestamp(issue['closed_at']) > after:
return True
return False
def landing20(issue):
"""Returns True iff this issue is marked landing-2.0."""
labels = issue.get('labels', [])
return any(label['name'] == 'landing-2.0' for label in labels)
def relevent_issue(issue, after):
"""Returns True iff this issue is something we should show in the changelog."""
return (closed_issue(issue, after) and
issue_completed(issue) and
issue_section(issue) and
not landing20(issue))
def relevant_issues(issues, after):
"""Yields relevant closed issues (closed after a given datetime) given a list of issues."""
logging.info('finding relevant issues after {}...'.format(after))
seen = set()
for issue in issues:
if relevent_issue(issue, after) and issue['title'] not in seen:
seen.add(issue['title'])
yield issue
def closed_issues(issues, after):
"""Yields closed issues (closed after a given datetime) given a list of issues."""
logging.info('finding closed issues after {}...'.format(after))
seen = set()
for issue in issues:
if closed_issue(issue, after) and issue['title'] not in seen:
seen.add(issue['title'])
yield issue
def all_issues(issues):
"""Yields unique set of issues given a list of issues."""
logging.info('finding issues...')
seen = set()
for issue in issues:
if issue['title'] not in seen:
seen.add(issue['title'])
yield issue
#######################################
# GitHub API
#######################################
def get_labels_url():
"""Returns github API URL for querying labels."""
return '{base_url}/{owner}/{repo}/labels'.format(**API_PARAMS)
def get_issues_url(page, after):
"""Returns github API URL for querying tags."""
template = '{base_url}/{owner}/{repo}/issues?state=closed&per_page=100&page={page}&since={after}'
return template.format(page=page, after=after.isoformat(), **API_PARAMS)
def get_tags_url():
"""Returns github API URL for querying tags."""
return '{base_url}/{owner}/{repo}/tags'.format(**API_PARAMS)
def parse_timestamp(timestamp):
"""Parse ISO8601 timestamps given by github API."""
dt = dateutil.parser.parse(timestamp)
return dt.astimezone(dateutil.tz.tzutc())
def read_url(url):
"""Reads given URL as JSON and returns data as loaded python object."""
logging.debug('reading {url} ...'.format(url=url))
token = os.environ.get("BOKEH_GITHUB_API_TOKEN")
headers = {}
if token:
headers['Authorization'] = 'token %s' % token
request = Request(url, headers=headers)
response = urlopen(request).read()
return json.loads(response.decode("UTF-8"))
def query_tags():
"""Hits the github API for repository tags and returns the data."""
return read_url(get_tags_url())
def query_issues(page, after):
"""Hits the github API for a single page of closed issues and returns the data."""
return read_url(get_issues_url(page, after))
def query_all_issues(after):
"""Hits the github API for all closed issues after the given date, returns the data."""
page = count(1)
data = []
while True:
page_data = query_issues(next(page), after)
if not page_data:
break
data.extend(page_data)
return data
def dateof(tag_name, tags):
"""Given a list of tags, returns the datetime of the tag with the given name; Otherwise None."""
for tag in tags:
if tag['name'] == tag_name:
commit = read_url(tag['commit']['url'])
return parse_timestamp(commit['commit']['committer']['date'])
return None
def get_data(query_func, load_data=False, save_data=False):
"""Gets data from query_func, optionally saving that data to a file; or loads data from a file."""
if hasattr(query_func, '__name__'):
func_name = query_func.__name__
elif hasattr(query_func, 'func'):
func_name = query_func.func.__name__
pickle_file = '{}.pickle'.format(func_name)
if load_data:
data = load_object(pickle_file)
else:
data = query_func()
if save_data:
save_object(pickle_file, data)
return data
#######################################
# Validation
#######################################
def check_issue(issue, after):
have_warnings = False
labels = issue.get('labels', [])
if 'pull_request' in issue:
if not any(label['name'].startswith('status: ') for label in labels):
logging.warning('pull request without status label: {}'.format(issue_line(issue)))
have_warnings = True
else:
if not any(label['name'].startswith('type: ') for label in labels):
if not any(label['name']=="reso: duplicate" for label in labels):
logging.warning('issue with no type label: {}'.format(issue_line((issue))))
have_warnings = True
if closed_issue(issue, after):
if not any(label['name'].startswith('reso: ') for label in labels):
if not any(label['name'] in IGNORE_ISSUE_TYPE for label in labels):
logging.warning('closed issue with no reso label: {}'.format(issue_line((issue))))
have_warnings = True
return have_warnings
def check_issues(issues, after=None):
"""Checks issues for BEP 1 compliance."""
issues = closed_issues(issues, after) if after else all_issues(issues)
issues = sorted(issues, key=ISSUES_SORT_KEY)
have_warnings = False
for section, issue_group in groupby(issues, key=ISSUES_BY_SECTION):
for issue in issue_group:
have_warnings |= check_issue(issue, after)
return have_warnings
#######################################
# Changelog
#######################################
def issue_line(issue):
"""Returns log line for given issue."""
template = '#{number} {tags}{title}'
tags = issue_tags(issue)
params = {
'title': issue['title'].capitalize().rstrip('.'),
'number': issue['number'],
'tags': ' '.join('[{}]'.format(tag) for tag in tags) + (' ' if tags else '')
}
return template.format(**params)
def generate_changelog(issues, after, heading, rtag=False):
"""Prints out changelog."""
relevent = relevant_issues(issues, after)
relevent = sorted(relevent, key=ISSUES_BY_SECTION)
def write(func, endofline="", append=""):
func(heading + '\n' + '-' * 20 + endofline)
for section, issue_group in groupby(relevent, key=ISSUES_BY_SECTION):
func(' * {}:'.format(section) + endofline)
for issue in reversed(list(issue_group)):
func(' - {}'.format(issue_line(issue)) + endofline)
func(endofline + append)
if rtag is not False:
with open("../CHANGELOG", "r+") as f:
content = f.read()
f.seek(0)
write(f.write, '\n', content)
else:
write(print)
if __name__ == '__main__':
parser = argparse.ArgumentParser(description='Creates a bokeh changelog using the github API.')
limit_group = parser.add_mutually_exclusive_group(required=True)
limit_group.add_argument('-d', '--since-date', metavar='DATE',
help='select issues that occurred after the given ISO8601 date')
limit_group.add_argument('-p', '--since-tag', metavar='TAG',
help='select issues that occurred after the given git tag')
parser.add_argument('-c', '--check', action='store_true', default=False,
help='check closed issues for BEP 1 compliance')
parser.add_argument('-r', '--release-tag', metavar='RELEASE',
help='the proposed new release tag.\n'
'NOTE: this will automatically write the output to the CHANGELOG')
data_group = parser.add_mutually_exclusive_group()
data_group.add_argument('-s', '--save-data', action='store_true', default=False,
help='save api query result data; useful for testing')
data_group.add_argument('-l', '--load-data', action='store_true', default=False,
help='load api data from previously saved data; useful for testing')
args = parser.parse_args()
if args.since_tag:
tags = get_data(query_tags, load_data=args.load_data, save_data=args.save_data)
after = dateof(args.since_tag, tags)
heading = 'Since {:>14}:'.format(args.since_tag)
elif args.since_date:
after = dateutil.parser.parse(args.since_date)
after = after.replace(tzinfo=dateutil.tz.tzlocal())
heading = 'Since {:>14}:'.format(after.date().isoformat())
issues = get_data(partial(query_all_issues, after), load_data=args.load_data, save_data=args.save_data)
if args.check:
have_warnings = check_issues(issues)
if have_warnings:
sys.exit(1)
sys.exit(0)
if args.release_tag:
heading = '{} {:>8}:'.format(str(datetime.date.today()), args.release_tag)
generate_changelog(issues, after, heading, args.release_tag)
else:
generate_changelog(issues, after, heading)
| {
"content_hash": "9398b49e988f685172a993807f194bcb",
"timestamp": "",
"source": "github",
"line_count": 367,
"max_line_length": 118,
"avg_line_length": 34.39509536784741,
"alnum_prop": 0.5983522142121525,
"repo_name": "timsnyder/bokeh",
"id": "881b5c26b3c4676ae4b5318908a1f6dd382fb0e7",
"size": "12646",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "scripts/issues.py",
"mode": "33261",
"license": "bsd-3-clause",
"language": [
{
"name": "Batchfile",
"bytes": "1442"
},
{
"name": "CSS",
"bytes": "24877"
},
{
"name": "Dockerfile",
"bytes": "4099"
},
{
"name": "HTML",
"bytes": "54062"
},
{
"name": "JavaScript",
"bytes": "27797"
},
{
"name": "Makefile",
"bytes": "886"
},
{
"name": "PowerShell",
"bytes": "713"
},
{
"name": "Python",
"bytes": "3827067"
},
{
"name": "Roff",
"bytes": "495"
},
{
"name": "Shell",
"bytes": "9953"
},
{
"name": "TypeScript",
"bytes": "2145262"
}
],
"symlink_target": ""
} |
sent('go to flight deck').
sent('move to flight deck').
sent('measure temperature').
sent('what is the pressure').
sent('is the fan switched on').
sent('is the fan at crew hatch switched on').
sent('is the fan switched on at crew hatch').
sent('switch on the fan').
sent('switch off the fan').
sent('switch it on').
sent('switch on the fan at crew hatch').
sent('open the door').
sent('close the door').
sent('is the door open').
sent('is the door closed').
sent('is the pressure increasing').
sent('is the pressure decreasing').
sent('the radiation').
sent('the temperature and pressure').
sent('pilots seat and crew hatch').
sent('pilots seat commanders seat and crew hatch').
sent('what are temperature and pressure').
sent('are temperature and pressure decreasing').
sent('switch them on').
sent('pilots seat').
sent('commanders seat').
sent('crew hatch').
sent('sleep stations').
sent('storage lockers').
sent('access ladder').
sent('oxygen').
sent('carbon dioxide').
| {
"content_hash": "14bdf08db4b21a949ca6868a8349f2b3",
"timestamp": "",
"source": "github",
"line_count": 40,
"max_line_length": 51,
"avg_line_length": 24.525,
"alnum_prop": 0.7054026503567788,
"repo_name": "TeamSPoon/logicmoo_workspace",
"id": "a1495adf61e249cb06d42ca328db7bffc5d4524e",
"size": "981",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "packs_sys/logicmoo_nlu/ext/regulus/doc/ACL2003/Experiments/corpora/psa_sents_small.pl",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "Batchfile",
"bytes": "342"
},
{
"name": "C",
"bytes": "1"
},
{
"name": "C++",
"bytes": "1"
},
{
"name": "CSS",
"bytes": "126627"
},
{
"name": "HTML",
"bytes": "839172"
},
{
"name": "Java",
"bytes": "11116"
},
{
"name": "JavaScript",
"bytes": "238700"
},
{
"name": "PHP",
"bytes": "42253"
},
{
"name": "Perl 6",
"bytes": "23"
},
{
"name": "Prolog",
"bytes": "440882"
},
{
"name": "PureBasic",
"bytes": "1334"
},
{
"name": "Rich Text Format",
"bytes": "3436542"
},
{
"name": "Roff",
"bytes": "42"
},
{
"name": "Shell",
"bytes": "61603"
},
{
"name": "TeX",
"bytes": "99504"
}
],
"symlink_target": ""
} |
/* eslint-disable react/prop-types */
/*
* Copyright (C) 2017-2019 Dremio Corporation
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import * as React from "react";
import clsx from "clsx";
import { forwardRef, type ReactNode } from "react";
type CardProps = {
children: ReactNode;
title?: JSX.Element;
toolbar?: JSX.Element;
className?: string;
};
export const Card = forwardRef<HTMLDivElement, CardProps>((props, ref) => {
const { children, className, title, toolbar, ...rest } = props;
return (
<article ref={ref} {...rest} className={clsx("dremio-card", className)}>
<header className="dremio-card__header">
{title && <span className="dremio-card__title">{title}</span>}
{toolbar && <div className="dremio-card__toolbar">{toolbar}</div>}
</header>
<div className="dremio-card__body">{children}</div>
</article>
);
});
| {
"content_hash": "0e9ced69fbc913c8d2df156a3c9767d7",
"timestamp": "",
"source": "github",
"line_count": 41,
"max_line_length": 76,
"avg_line_length": 34.09756097560975,
"alnum_prop": 0.6838340486409156,
"repo_name": "dremio/dremio-oss",
"id": "41d97d935abadfcca4e802dea9fd8c7c6266b8f0",
"size": "1398",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "dac/ui-lib/components/Card/Card.tsx",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "CSS",
"bytes": "47376"
},
{
"name": "Dockerfile",
"bytes": "1668"
},
{
"name": "FreeMarker",
"bytes": "132156"
},
{
"name": "GAP",
"bytes": "15936"
},
{
"name": "HTML",
"bytes": "6544"
},
{
"name": "Java",
"bytes": "39679012"
},
{
"name": "JavaScript",
"bytes": "5439822"
},
{
"name": "Less",
"bytes": "547002"
},
{
"name": "SCSS",
"bytes": "95688"
},
{
"name": "Shell",
"bytes": "16063"
},
{
"name": "TypeScript",
"bytes": "887739"
}
],
"symlink_target": ""
} |
package org.elasticsearch.gradle;
import org.gradle.api.tasks.WorkResult;
/**
* An interface for tasks that support basic file operations.
* Methods will be added as needed.
*/
public interface FileSystemOperationsAware {
WorkResult delete(Object... objects);
}
| {
"content_hash": "a2ad6ec66568df9ecd4bf9cdfc6586de",
"timestamp": "",
"source": "github",
"line_count": 13,
"max_line_length": 61,
"avg_line_length": 21,
"alnum_prop": 0.7545787545787546,
"repo_name": "nknize/elasticsearch",
"id": "b082b86e6c2bb0270f932af7d68ca19c0fdc120e",
"size": "1062",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "buildSrc/src/main/java/org/elasticsearch/gradle/FileSystemOperationsAware.java",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "ANTLR",
"bytes": "12298"
},
{
"name": "Batchfile",
"bytes": "16353"
},
{
"name": "Emacs Lisp",
"bytes": "3341"
},
{
"name": "FreeMarker",
"bytes": "45"
},
{
"name": "Groovy",
"bytes": "251795"
},
{
"name": "HTML",
"bytes": "5348"
},
{
"name": "Java",
"bytes": "36849935"
},
{
"name": "Perl",
"bytes": "7116"
},
{
"name": "Python",
"bytes": "76127"
},
{
"name": "Shell",
"bytes": "102829"
}
],
"symlink_target": ""
} |
package net.md_5.bungee.connection;
import com.google.common.base.Charsets;
import com.google.common.base.Preconditions;
import java.math.BigInteger;
import java.net.InetSocketAddress;
import java.net.URLEncoder;
import java.security.MessageDigest;
import java.util.ArrayList;
import java.util.List;
import java.util.UUID;
import java.util.logging.Level;
import javax.crypto.SecretKey;
import com.google.gson.Gson;
import java.util.concurrent.TimeUnit;
import lombok.Getter;
import lombok.RequiredArgsConstructor;
import net.md_5.bungee.*;
import net.md_5.bungee.api.Callback;
import net.md_5.bungee.api.ChatColor;
import net.md_5.bungee.api.Favicon;
import net.md_5.bungee.api.ProxyServer;
import net.md_5.bungee.api.ServerPing;
import net.md_5.bungee.api.chat.BaseComponent;
import net.md_5.bungee.api.chat.TextComponent;
import net.md_5.bungee.api.config.ListenerInfo;
import net.md_5.bungee.api.config.ServerInfo;
import net.md_5.bungee.api.connection.PendingConnection;
import net.md_5.bungee.api.connection.ProxiedPlayer;
import net.md_5.bungee.api.event.LoginEvent;
import net.md_5.bungee.api.event.PostLoginEvent;
import net.md_5.bungee.api.event.ProxyPingEvent;
import net.md_5.bungee.chat.ComponentSerializer;
import net.md_5.bungee.http.HttpClient;
import net.md_5.bungee.netty.HandlerBoss;
import net.md_5.bungee.netty.ChannelWrapper;
import net.md_5.bungee.netty.PacketHandler;
import net.md_5.bungee.netty.PipelineUtils;
import net.md_5.bungee.netty.cipher.CipherDecoder;
import net.md_5.bungee.netty.cipher.CipherEncoder;
import net.md_5.bungee.protocol.DefinedPacket;
import net.md_5.bungee.protocol.packet.Handshake;
import net.md_5.bungee.protocol.packet.PluginMessage;
import net.md_5.bungee.protocol.packet.EncryptionResponse;
import net.md_5.bungee.protocol.packet.EncryptionRequest;
import net.md_5.bungee.protocol.packet.Kick;
import net.md_5.bungee.api.AbstractReconnectHandler;
import net.md_5.bungee.api.event.PlayerHandshakeEvent;
import net.md_5.bungee.api.event.PreLoginEvent;
import net.md_5.bungee.jni.cipher.BungeeCipher;
import net.md_5.bungee.protocol.Protocol;
import net.md_5.bungee.protocol.ProtocolConstants;
import net.md_5.bungee.protocol.packet.LegacyHandshake;
import net.md_5.bungee.protocol.packet.LegacyPing;
import net.md_5.bungee.protocol.packet.LoginRequest;
import net.md_5.bungee.protocol.packet.LoginSuccess;
import net.md_5.bungee.protocol.packet.PingPacket;
import net.md_5.bungee.protocol.packet.StatusRequest;
import net.md_5.bungee.protocol.packet.StatusResponse;
import net.md_5.bungee.util.BoundedArrayList;
@RequiredArgsConstructor
public class InitialHandler extends PacketHandler implements PendingConnection
{
private final ProxyServer bungee;
private ChannelWrapper ch;
@Getter
private final ListenerInfo listener;
@Getter
private Handshake handshake;
@Getter
private LoginRequest loginRequest;
private EncryptionRequest request;
@Getter
private final List<PluginMessage> registerMessages = new BoundedArrayList<>( 128 );
private State thisState = State.HANDSHAKE;
private final Unsafe unsafe = new Unsafe()
{
@Override
public void sendPacket(DefinedPacket packet)
{
ch.write( packet );
}
};
@Getter
private boolean onlineMode = BungeeCord.getInstance().config.isOnlineMode();
@Getter
private InetSocketAddress virtualHost;
@Getter
private UUID uniqueId;
@Getter
private UUID offlineId;
@Getter
private LoginResult loginProfile;
@Getter
private boolean legacy;
@Getter
private String extraDataInHandshake = "";
private enum State
{
HANDSHAKE, STATUS, PING, USERNAME, ENCRYPT, FINISHED;
}
@Override
public void connected(ChannelWrapper channel) throws Exception
{
this.ch = channel;
}
@Override
public void exception(Throwable t) throws Exception
{
disconnect( ChatColor.RED + Util.exception( t ) );
}
@Override
public void handle(PluginMessage pluginMessage) throws Exception
{
// TODO: Unregister?
if ( pluginMessage.getTag().equals( "REGISTER" ) )
{
registerMessages.add( pluginMessage );
}
}
@Override
public void handle(LegacyHandshake legacyHandshake) throws Exception
{
this.legacy = true;
ch.getHandle().writeAndFlush( bungee.getTranslation( "outdated_client" ) );
ch.close();
}
@Override
public void handle(LegacyPing ping) throws Exception
{
this.legacy = true;
final boolean v1_5 = ping.isV1_5();
ServerPing legacy = new ServerPing( new ServerPing.Protocol( bungee.getName() + " " + bungee.getGameVersion(), bungee.getProtocolVersion() ),
new ServerPing.Players( listener.getMaxPlayers(), bungee.getOnlineCount(), null ),
new TextComponent( TextComponent.fromLegacyText( listener.getMotd() ) ), (Favicon) null );
Callback<ProxyPingEvent> callback = new Callback<ProxyPingEvent>()
{
@Override
public void done(ProxyPingEvent result, Throwable error)
{
if ( ch.isClosed() )
{
return;
}
ServerPing legacy = result.getResponse();
String kickMessage;
if ( v1_5 )
{
kickMessage = ChatColor.DARK_BLUE
+ "\00" + 127
+ '\00' + legacy.getVersion().getName()
+ '\00' + getFirstLine( legacy.getDescription() )
+ '\00' + legacy.getPlayers().getOnline()
+ '\00' + legacy.getPlayers().getMax();
} else
{
// Clients <= 1.3 don't support colored motds because the color char is used as delimiter
kickMessage = ChatColor.stripColor( getFirstLine( legacy.getDescription() ) )
+ '\u00a7' + legacy.getPlayers().getOnline()
+ '\u00a7' + legacy.getPlayers().getMax();
}
ch.getHandle().writeAndFlush( kickMessage );
ch.close();
}
};
bungee.getPluginManager().callEvent( new ProxyPingEvent( this, legacy, callback ) );
}
private static String getFirstLine(String str)
{
int pos = str.indexOf( '\n' );
return pos == -1 ? str : str.substring( 0, pos );
}
@Override
public void handle(StatusRequest statusRequest) throws Exception
{
Preconditions.checkState( thisState == State.STATUS, "Not expecting STATUS" );
ServerInfo forced = AbstractReconnectHandler.getForcedHost( this );
final String motd = ( forced != null ) ? forced.getMotd() : listener.getMotd();
Callback<ServerPing> pingBack = new Callback<ServerPing>()
{
@Override
public void done(ServerPing result, Throwable error)
{
if ( error != null )
{
result = new ServerPing();
result.setDescription( bungee.getTranslation( "ping_cannot_connect" ) );
bungee.getLogger().log( Level.WARNING, "Error pinging remote server", error );
}
Callback<ProxyPingEvent> callback = new Callback<ProxyPingEvent>()
{
@Override
public void done(ProxyPingEvent pingResult, Throwable error)
{
BungeeCord.getInstance().getConnectionThrottle().unthrottle( getAddress().getAddress() );
Gson gson = BungeeCord.getInstance().gson;
unsafe.sendPacket( new StatusResponse( gson.toJson( pingResult.getResponse() ) ) );
}
};
bungee.getPluginManager().callEvent( new ProxyPingEvent( InitialHandler.this, result, callback ) );
}
};
if ( forced != null && listener.isPingPassthrough() )
{
( (BungeeServerInfo) forced ).ping( pingBack, handshake.getProtocolVersion() );
} else
{
int protocol = ( Protocol.supportedVersions.contains( handshake.getProtocolVersion() ) ) ? handshake.getProtocolVersion() : bungee.getProtocolVersion();
pingBack.done( new ServerPing(
new ServerPing.Protocol( bungee.getName() + " " + bungee.getGameVersion(), protocol ),
new ServerPing.Players( listener.getMaxPlayers(), bungee.getOnlineCount(), null ),
motd, BungeeCord.getInstance().config.getFaviconObject() ),
null );
}
thisState = State.PING;
}
@Override
public void handle(PingPacket ping) throws Exception
{
Preconditions.checkState( thisState == State.PING, "Not expecting PING" );
unsafe.sendPacket( ping );
disconnect( "" );
}
@Override
public void handle(Handshake handshake) throws Exception
{
Preconditions.checkState( thisState == State.HANDSHAKE, "Not expecting HANDSHAKE" );
this.handshake = handshake;
ch.setVersion( handshake.getProtocolVersion() );
// Starting with FML 1.8, a "\0FML\0" token is appended to the handshake. This interferes
// with Bungee's IP forwarding, so we detect it, and remove it from the host string, for now.
// We know FML appends \00FML\00. However, we need to also consider that other systems might
// add their own data to the end of the string. So, we just take everything from the \0 character
// and save it for later.
if ( handshake.getHost().contains( "\0" ) )
{
String[] split = handshake.getHost().split( "\0", 2 );
handshake.setHost( split[0] );
extraDataInHandshake = "\0" + split[1];
}
// SRV records can end with a . depending on DNS / client.
if ( handshake.getHost().endsWith( "." ) )
{
handshake.setHost( handshake.getHost().substring( 0, handshake.getHost().length() - 1 ) );
}
this.virtualHost = InetSocketAddress.createUnresolved( handshake.getHost(), handshake.getPort() );
bungee.getLogger().log( Level.INFO, "{0} has connected", this );
bungee.getPluginManager().callEvent( new PlayerHandshakeEvent( InitialHandler.this, handshake ) );
switch ( handshake.getRequestedProtocol() )
{
case 1:
// Ping
thisState = State.STATUS;
ch.setProtocol( Protocol.STATUS );
break;
case 2:
thisState = State.USERNAME;
ch.setProtocol( Protocol.LOGIN );
// Login
break;
default:
throw new IllegalArgumentException( "Cannot request protocol " + handshake.getRequestedProtocol() );
}
}
@Override
public void handle(LoginRequest loginRequest) throws Exception
{
Preconditions.checkState( thisState == State.USERNAME, "Not expecting USERNAME" );
this.loginRequest = loginRequest;
if ( !Protocol.supportedVersions.contains( handshake.getProtocolVersion() ) )
{
disconnect( bungee.getTranslation( "outdated_server" ) );
return;
}
if ( getName().contains( "." ) )
{
disconnect( bungee.getTranslation( "name_invalid" ) );
return;
}
if ( getName().length() > 16 )
{
disconnect( bungee.getTranslation( "name_too_long" ) );
return;
}
int limit = BungeeCord.getInstance().config.getPlayerLimit();
if ( limit > 0 && bungee.getOnlineCount() > limit )
{
disconnect( bungee.getTranslation( "proxy_full" ) );
return;
}
// If offline mode and they are already on, don't allow connect
// We can just check by UUID here as names are based on UUID
if ( !isOnlineMode() && bungee.getPlayer( getUniqueId() ) != null )
{
disconnect( bungee.getTranslation( "already_connected_proxy" ) );
return;
}
Callback<PreLoginEvent> callback = new Callback<PreLoginEvent>()
{
@Override
public void done(PreLoginEvent result, Throwable error)
{
if ( result.isCancelled() )
{
disconnect( result.getCancelReason() );
return;
}
if ( ch.isClosed() )
{
return;
}
if ( onlineMode )
{
unsafe().sendPacket( request = EncryptionUtil.encryptRequest() );
} else
{
finish();
}
thisState = State.ENCRYPT;
}
};
// fire pre login event
bungee.getPluginManager().callEvent( new PreLoginEvent( InitialHandler.this, callback ) );
}
@Override
public void handle(final EncryptionResponse encryptResponse) throws Exception
{
Preconditions.checkState( thisState == State.ENCRYPT, "Not expecting ENCRYPT" );
SecretKey sharedKey = EncryptionUtil.getSecret( encryptResponse, request );
BungeeCipher decrypt = EncryptionUtil.getCipher( false, sharedKey );
ch.addBefore( PipelineUtils.FRAME_DECODER, PipelineUtils.DECRYPT_HANDLER, new CipherDecoder( decrypt ) );
BungeeCipher encrypt = EncryptionUtil.getCipher( true, sharedKey );
ch.addBefore( PipelineUtils.FRAME_PREPENDER, PipelineUtils.ENCRYPT_HANDLER, new CipherEncoder( encrypt ) );
String encName = URLEncoder.encode( InitialHandler.this.getName(), "UTF-8" );
MessageDigest sha = MessageDigest.getInstance( "SHA-1" );
for ( byte[] bit : new byte[][]
{
request.getServerId().getBytes( "ISO_8859_1" ), sharedKey.getEncoded(), EncryptionUtil.keys.getPublic().getEncoded()
} )
{
sha.update( bit );
}
String encodedHash = URLEncoder.encode( new BigInteger( sha.digest() ).toString( 16 ), "UTF-8" );
String authURL = "https://sessionserver.mojang.com/session/minecraft/hasJoined?username=" + encName + "&serverId=" + encodedHash;
Callback<String> handler = new Callback<String>()
{
@Override
public void done(String result, Throwable error)
{
if ( error == null )
{
LoginResult obj = BungeeCord.getInstance().gson.fromJson( result, LoginResult.class );
if ( obj != null )
{
loginProfile = obj;
uniqueId = Util.getUUID( obj.getId() );
finish();
return;
}
disconnect( "Not authenticated with Minecraft.net" );
} else
{
disconnect( bungee.getTranslation( "mojang_fail" ) );
bungee.getLogger().log( Level.SEVERE, "Error authenticating " + getName() + " with minecraft.net", error );
}
}
};
HttpClient.get( authURL, ch.getHandle().eventLoop(), handler );
}
private void finish()
{
if ( isOnlineMode() )
{
// Check for multiple connections
// We have to check for the old name first
ProxiedPlayer oldName = bungee.getPlayer( getName() );
if ( oldName != null )
{
// TODO See #1218
oldName.disconnect( bungee.getTranslation( "already_connected_proxy" ) );
}
// And then also for their old UUID
ProxiedPlayer oldID = bungee.getPlayer( getUniqueId() );
if ( oldID != null )
{
// TODO See #1218
oldID.disconnect( bungee.getTranslation( "already_connected_proxy" ) );
}
} else
{
// In offline mode the existing user stays and we kick the new one
ProxiedPlayer oldName = bungee.getPlayer( getName() );
if ( oldName != null )
{
// TODO See #1218
disconnect( bungee.getTranslation( "already_connected_proxy" ) );
return;
}
}
offlineId = java.util.UUID.nameUUIDFromBytes( ( "OfflinePlayer:" + getName() ).getBytes( Charsets.UTF_8 ) );
if ( uniqueId == null )
{
uniqueId = offlineId;
}
Callback<LoginEvent> complete = new Callback<LoginEvent>()
{
@Override
public void done(LoginEvent result, Throwable error)
{
if ( result.isCancelled() )
{
disconnect( result.getCancelReason() );
return;
}
if ( ch.isClosed() )
{
return;
}
ch.getHandle().eventLoop().execute( new Runnable()
{
@Override
public void run()
{
if ( ch.getHandle().isActive() )
{
UserConnection userCon = new UserConnection( bungee, ch, getName(), InitialHandler.this );
userCon.setCompressionThreshold( BungeeCord.getInstance().config.getCompressionThreshold() );
userCon.init();
unsafe.sendPacket( new LoginSuccess( getUniqueId().toString(), getName() ) ); // With dashes in between
ch.setProtocol( Protocol.GAME );
ch.getHandle().pipeline().get( HandlerBoss.class ).setHandler( new UpstreamBridge( bungee, userCon ) );
bungee.getPluginManager().callEvent( new PostLoginEvent( userCon ) );
ServerInfo server;
if ( bungee.getReconnectHandler() != null )
{
server = bungee.getReconnectHandler().getServer( userCon );
} else
{
server = AbstractReconnectHandler.getForcedHost( InitialHandler.this );
}
if ( server == null )
{
server = bungee.getServerInfo( listener.getDefaultServer() );
}
userCon.connect( server, null, true );
thisState = State.FINISHED;
}
}
} );
}
};
// fire login event
bungee.getPluginManager().callEvent( new LoginEvent( InitialHandler.this, complete ) );
}
@Override
public void disconnect(String reason)
{
disconnect( TextComponent.fromLegacyText( reason ) );
}
@Override
public void disconnect(final BaseComponent... reason)
{
if ( !ch.isClosed() )
{
// Why do we have to delay this you might ask? Well the simple reason is MOJANG.
// Despite many a bug report posted, ever since the 1.7 protocol rewrite, the client STILL has a race condition upon switching protocols.
// As such, despite the protocol switch packets already having been sent, there is the possibility of a client side exception
// To help combat this we will wait half a second before actually sending the disconnected packet so that whoever is on the other
// end has a somewhat better chance of receiving the proper packet.
ch.getHandle().eventLoop().schedule( new Runnable()
{
@Override
public void run()
{
if ( thisState != State.STATUS && thisState != State.PING )
{
unsafe().sendPacket( new Kick( ComponentSerializer.toString( reason ) ) );
}
ch.close();
}
}, 500, TimeUnit.MILLISECONDS );
}
}
@Override
public void disconnect(BaseComponent reason)
{
disconnect( new BaseComponent[]
{
reason
} );
}
@Override
public String getName()
{
return ( loginRequest == null ) ? null : loginRequest.getData();
}
@Override
public int getVersion()
{
return ( handshake == null ) ? -1 : handshake.getProtocolVersion();
}
@Override
public InetSocketAddress getAddress()
{
return (InetSocketAddress) ch.getHandle().remoteAddress();
}
@Override
public Unsafe unsafe()
{
return unsafe;
}
@Override
public void setOnlineMode(boolean onlineMode)
{
Preconditions.checkState( thisState == State.USERNAME, "Can only set online mode status whilst state is username" );
this.onlineMode = onlineMode;
}
@Override
public void setUniqueId(UUID uuid)
{
Preconditions.checkState( thisState == State.USERNAME, "Can only set uuid while state is username" );
Preconditions.checkState( !onlineMode, "Can only set uuid when online mode is false" );
this.uniqueId = uuid;
}
@Override
public String getUUID()
{
return uniqueId.toString().replaceAll( "-", "" );
}
@Override
public String toString()
{
return "[" + ( ( getName() != null ) ? getName() : getAddress() ) + "] <-> InitialHandler";
}
@Override
public boolean isConnected()
{
return !ch.isClosed();
}
}
| {
"content_hash": "0ce2c5a61d22832836cf741b6799fdab",
"timestamp": "",
"source": "github",
"line_count": 610,
"max_line_length": 164,
"avg_line_length": 36.47868852459016,
"alnum_prop": 0.5720384684522739,
"repo_name": "LinEvil/BungeeCord",
"id": "34b1a4bcfc88b5a205963d68da6c655a093afb17",
"size": "22252",
"binary": false,
"copies": "2",
"ref": "refs/heads/master",
"path": "proxy/src/main/java/net/md_5/bungee/connection/InitialHandler.java",
"mode": "33188",
"license": "bsd-3-clause",
"language": [
{
"name": "C++",
"bytes": "4045"
},
{
"name": "Java",
"bytes": "567811"
},
{
"name": "Shell",
"bytes": "284"
}
],
"symlink_target": ""
} |
using System.Threading;
using System.Diagnostics;
using System.Security.Permissions;
using System.Runtime.InteropServices;
using System.Runtime.Serialization;
namespace System
{
/// <summary>
/// Lazy
/// </summary>
/// <typeparam name="T"></typeparam>
[Serializable, DebuggerDisplay("ThreadSafetyMode={Mode}, IsValueCreated={IsValueCreated}, IsValueFaulted={IsValueFaulted}, Value={ValueForDebugDisplay}"), DebuggerTypeProxy(typeof(System_LazyDebugView<>)), ComVisible(false), HostProtection(SecurityAction.LinkDemand, Synchronization = true, ExternalThreading = true)]
public class Lazy<T>
{
private volatile object _boxed;
[NonSerialized]
private readonly object _threadSafeObj;
[NonSerialized]
private Func<T> m_valueFactory;
private static Func<T> PUBLICATION_ONLY_OR_ALREADY_INITIALIZED = (() => default(T));
static Lazy() { }
//[TargetedPatchingOptOut("Performance critical to inline this type of method across NGen image boundaries")]
/// <summary>
/// Initializes a new instance of the <see cref="Lazy<T>"/> class.
/// </summary>
public Lazy()
: this(LazyThreadSafetyMode.ExecutionAndPublication) { }
/// <summary>
/// Initializes a new instance of the <see cref="Lazy<T>"/> class.
/// </summary>
/// <param name="isThreadSafe">if set to <c>true</c> [is thread safe].</param>
public Lazy(bool isThreadSafe)
: this(isThreadSafe ? LazyThreadSafetyMode.ExecutionAndPublication : LazyThreadSafetyMode.None) { }
//[TargetedPatchingOptOut("Performance critical to inline this type of method across NGen image boundaries")]
/// <summary>
/// Initializes a new instance of the <see cref="Lazy<T>"/> class.
/// </summary>
/// <param name="valueFactory">The value factory.</param>
public Lazy(Func<T> valueFactory)
: this(valueFactory, LazyThreadSafetyMode.ExecutionAndPublication) { }
/// <summary>
/// Initializes a new instance of the <see cref="Lazy<T>"/> class.
/// </summary>
/// <param name="mode">The mode.</param>
public Lazy(LazyThreadSafetyMode mode)
{
_threadSafeObj = GetObjectFromMode(mode);
}
/// <summary>
/// Initializes a new instance of the <see cref="Lazy<T>"/> class.
/// </summary>
/// <param name="valueFactory">The value factory.</param>
/// <param name="isThreadSafe">if set to <c>true</c> [is thread safe].</param>
public Lazy(Func<T> valueFactory, bool isThreadSafe)
: this(valueFactory, isThreadSafe ? LazyThreadSafetyMode.ExecutionAndPublication : LazyThreadSafetyMode.None) { }
/// <summary>
/// Initializes a new instance of the <see cref="Lazy<T>"/> class.
/// </summary>
/// <param name="valueFactory">The value factory.</param>
/// <param name="mode">The mode.</param>
public Lazy(Func<T> valueFactory, LazyThreadSafetyMode mode)
{
if (valueFactory == null)
throw new ArgumentNullException("valueFactory");
_threadSafeObj = GetObjectFromMode(mode);
m_valueFactory = valueFactory;
}
private Boxed CreateValue()
{
Boxed boxed = null;
LazyThreadSafetyMode mode = Mode;
if (m_valueFactory != null)
{
try
{
if ((mode != LazyThreadSafetyMode.PublicationOnly) && (m_valueFactory == PUBLICATION_ONLY_OR_ALREADY_INITIALIZED))
throw new InvalidOperationException("Lazy_Value_RecursiveCallsToValue");
var valueFactory = m_valueFactory;
if (mode != LazyThreadSafetyMode.PublicationOnly)
m_valueFactory = PUBLICATION_ONLY_OR_ALREADY_INITIALIZED;
return new Boxed(valueFactory());
}
catch (Exception exception)
{
if (mode != LazyThreadSafetyMode.PublicationOnly)
_boxed = new LazyInternalExceptionHolder(exception.PrepareForRethrow());
throw;
}
}
try { boxed = new Boxed((T)Activator.CreateInstance(typeof(T))); }
catch (MissingMethodException)
{
Exception ex = new MissingMemberException("Lazy_CreateValue_NoParameterlessCtorForT");
if (mode != LazyThreadSafetyMode.PublicationOnly)
_boxed = new LazyInternalExceptionHolder(ex);
throw ex;
}
return boxed;
}
private static object GetObjectFromMode(LazyThreadSafetyMode mode)
{
if (mode == LazyThreadSafetyMode.ExecutionAndPublication)
return new object();
if (mode == LazyThreadSafetyMode.PublicationOnly)
return PUBLICATION_ONLY_OR_ALREADY_INITIALIZED;
if (mode != LazyThreadSafetyMode.None)
throw new ArgumentOutOfRangeException("mode", "Lazy_ctor_ModeInvalid");
return null;
}
private T LazyInitValue()
{
Boxed boxed = null;
switch (Mode)
{
case LazyThreadSafetyMode.None:
boxed = CreateValue();
_boxed = boxed;
break;
case LazyThreadSafetyMode.PublicationOnly:
boxed = CreateValue();
#pragma warning disable 420
if (Interlocked.CompareExchange(ref _boxed, boxed, null) != null)
boxed = (Boxed)_boxed;
#pragma warning restore 420
break;
default:
lock (_threadSafeObj)
{
if (_boxed == null)
{
boxed = CreateValue();
_boxed = boxed;
}
else
{
boxed = (_boxed as Boxed);
if (boxed == null)
{
var holder = (_boxed as LazyInternalExceptionHolder);
throw holder._exception;
}
}
}
break;
}
return boxed._value;
}
[OnSerializing]
private void OnSerializing(StreamingContext context)
{
T local1 = Value;
}
/// <summary>
/// Returns a <see cref="System.String"/> that represents this instance.
/// </summary>
/// <returns>
/// A <see cref="System.String"/> that represents this instance.
/// </returns>
public override string ToString()
{
if (!IsValueCreated)
return "Lazy_ToString_ValueNotCreated";
return this.Value.ToString();
}
/// <summary>
/// Gets a value indicating whether this instance is value created.
/// </summary>
/// <value>
/// <c>true</c> if this instance is value created; otherwise, <c>false</c>.
/// </value>
public bool IsValueCreated
{
//[TargetedPatchingOptOut("Performance critical to inline across NGen image boundaries")]
get { return (_boxed != null && _boxed is Boxed); }
}
internal bool IsValueFaulted
{
get { return (_boxed is LazyInternalExceptionHolder); }
}
internal LazyThreadSafetyMode Mode
{
get
{
if (_threadSafeObj == null)
return LazyThreadSafetyMode.None;
if (_threadSafeObj == (object)PUBLICATION_ONLY_OR_ALREADY_INITIALIZED)
return LazyThreadSafetyMode.PublicationOnly;
return LazyThreadSafetyMode.ExecutionAndPublication;
}
}
/// <summary>
/// Gets the value.
/// </summary>
[DebuggerBrowsable(DebuggerBrowsableState.Never)]
public T Value
{
get
{
Boxed boxed = null;
if (_boxed != null)
{
boxed = (_boxed as Boxed);
if (boxed != null)
return boxed._value;
var holder = (_boxed as LazyInternalExceptionHolder);
throw holder._exception;
}
//Debugger.NotifyOfCrossThreadDependency();
return LazyInitValue();
}
}
internal T ValueForDebugDisplay
{
get
{
if (!this.IsValueCreated)
return default(T);
return ((Boxed)_boxed)._value;
}
}
[Serializable]
private class Boxed
{
internal T _value;
//[TargetedPatchingOptOut("Performance critical to inline this type of method across NGen image boundaries")]
internal Boxed(T value)
{
_value = value;
}
}
private class LazyInternalExceptionHolder
{
internal Exception _exception;
//[TargetedPatchingOptOut("Performance critical to inline this type of method across NGen image boundaries")]
internal LazyInternalExceptionHolder(Exception ex)
{
_exception = ex;
}
}
}
}
#endif | {
"content_hash": "1b60941052134775d1cce16fe807829a",
"timestamp": "",
"source": "github",
"line_count": 258,
"max_line_length": 321,
"avg_line_length": 39.3875968992248,
"alnum_prop": 0.5132847864593584,
"repo_name": "BclEx/BclEx-Abstract",
"id": "66ec59dfad9b86e433c6c99246a9fc07d56617f8",
"size": "10228",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "src/System.Abstract/+Polyfill/Lazy.cs",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "Batchfile",
"bytes": "1070"
},
{
"name": "C#",
"bytes": "2471042"
},
{
"name": "PowerShell",
"bytes": "39667"
}
],
"symlink_target": ""
} |
using System;
using System.Collections.Generic;
using System.Text;
using System.Windows.Forms;
using System.Drawing;
namespace XenAdmin.Controls
{
class PanelNoFocusScroll : Panel
{
object scrollLock = new object();
public bool defaultBehaviour = false;
protected override System.Drawing.Point ScrollToControl(Control activeControl)
{
if (defaultBehaviour)
return base.ScrollToControl(activeControl);
return DisplayRectangle.Location;
}
public void ForceScrollTo(Control c)
{
lock (scrollLock)
{
defaultBehaviour = true;
ScrollControlIntoView(c);
defaultBehaviour = false;
}
}
}
}
| {
"content_hash": "31538f91502b57b71dedf263a1b67e8c",
"timestamp": "",
"source": "github",
"line_count": 34,
"max_line_length": 86,
"avg_line_length": 23.176470588235293,
"alnum_prop": 0.5951776649746193,
"repo_name": "aftabahmedsajid/XenCenter-Complete-dependencies-",
"id": "e3f8eaff6fbcad5ec973a41db757117ca609a650",
"size": "2218",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "XenAdmin/Controls/PanelNoFocusScroll.cs",
"mode": "33188",
"license": "bsd-2-clause",
"language": [
{
"name": "C",
"bytes": "1907"
},
{
"name": "C#",
"bytes": "16370140"
},
{
"name": "C++",
"bytes": "21035"
},
{
"name": "JavaScript",
"bytes": "812"
},
{
"name": "PowerShell",
"bytes": "40"
},
{
"name": "Shell",
"bytes": "62958"
},
{
"name": "Visual Basic",
"bytes": "11351"
}
],
"symlink_target": ""
} |
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html><head><meta http-equiv="Content-Type" content="text/html;charset=UTF-8">
<title>Chinese Morphological Analyzer(Chen): /home/vernkin/projects/chinese-ma-chen/source/vsynonym/types.h Source File</title>
<link href="tabs.css" rel="stylesheet" type="text/css">
<link href="doxygen.css" rel="stylesheet" type="text/css">
</head><body>
<!-- Generated by Doxygen 1.5.8 -->
<div class="navigation" id="top">
<div class="tabs">
<ul>
<li><a href="index.html"><span>Main Page</span></a></li>
<li><a href="annotated.html"><span>Classes</span></a></li>
<li class="current"><a href="files.html"><span>Files</span></a></li>
</ul>
</div>
<div class="tabs">
<ul>
<li><a href="files.html"><span>File List</span></a></li>
<li><a href="globals.html"><span>File Members</span></a></li>
</ul>
</div>
<h1>/home/vernkin/projects/chinese-ma-chen/source/vsynonym/types.h</h1><div class="fragment"><pre class="fragment"><a name="l00001"></a>00001 <span class="preprocessor">#ifndef TYPES_H</span>
<a name="l00002"></a>00002 <span class="preprocessor"></span><span class="preprocessor">#define TYPES_H</span>
<a name="l00003"></a>00003 <span class="preprocessor"></span>
<a name="l00004"></a>00004 <span class="preprocessor">#ifndef WIN32</span>
<a name="l00005"></a>00005 <span class="preprocessor"></span><span class="preprocessor">#include <stddef.h></span>
<a name="l00006"></a>00006 <span class="preprocessor">#include <sys/types.h></span>
<a name="l00007"></a>00007 <span class="preprocessor">#include <stdint.h></span>
<a name="l00008"></a>00008 <span class="comment">/*#else</span>
<a name="l00009"></a>00009 <span class="comment">#include <sys/types.h></span>
<a name="l00010"></a>00010 <span class="comment">typedef signed char int8_t;</span>
<a name="l00011"></a>00011 <span class="comment">typedef short int16_t;</span>
<a name="l00012"></a>00012 <span class="comment">typedef long int32_t;</span>
<a name="l00013"></a>00013 <span class="comment">typedef __int64 int64_t;</span>
<a name="l00014"></a>00014 <span class="comment">typedef unsigned char uint8_t;</span>
<a name="l00015"></a>00015 <span class="comment">typedef unsigned short uint16_t;</span>
<a name="l00016"></a>00016 <span class="comment">typedef unsigned long uint32_t;</span>
<a name="l00017"></a>00017 <span class="comment">typedef unsigned __int64 uint64_t;</span>
<a name="l00018"></a>00018 <span class="comment">*/</span>
<a name="l00019"></a>00019 <span class="preprocessor">#endif //end of WIN32</span>
<a name="l00020"></a>00020 <span class="preprocessor"></span>
<a name="l00021"></a>00021 <span class="preprocessor">#endif // end of TYPES_H</span>
</pre></div></div>
<hr size="1"><address style="text-align: right;"><small>Generated on Fri Sep 25 15:20:06 2009 for Chinese Morphological Analyzer(Chen) by
<a href="http://www.doxygen.org/index.html">
<img src="doxygen.png" alt="doxygen" align="middle" border="0"></a> 1.5.8 </small></address>
</body>
</html>
| {
"content_hash": "2bcef11bed51c2a337be6f087f3d5f77",
"timestamp": "",
"source": "github",
"line_count": 48,
"max_line_length": 191,
"avg_line_length": 67.02083333333333,
"alnum_prop": 0.6540254895865714,
"repo_name": "izenecloud/icma",
"id": "bef56f8bbafc8755bb927d058a57070519650e59",
"size": "3217",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "docs/html/types_8h-source.html",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "C",
"bytes": "1892686"
},
{
"name": "C++",
"bytes": "855638"
},
{
"name": "CSS",
"bytes": "8463"
},
{
"name": "Objective-C",
"bytes": "17121"
},
{
"name": "Ruby",
"bytes": "608"
},
{
"name": "Shell",
"bytes": "272640"
},
{
"name": "TeX",
"bytes": "367572"
}
],
"symlink_target": ""
} |
activate :autoprefixer do |prefix|
prefix.browsers = "last 2 versions"
end
# Layouts
# https://middlemanapp.com/basics/layouts/
# Per-page layout changes
page '/*.xml', layout: false
page '/*.json', layout: false
page '/*.txt', layout: false
activate :i18n, :langs => [:en, :fr]
# With alternative layout
# page '/path/to/file.html', layout: 'other_layout'
# Proxy pages
# https://middlemanapp.com/advanced/dynamic-pages/
# proxy(
# '/this-page-has-no-template.html',
# '/template-file.html',
# locals: {
# which_fake_page: 'Rendering a fake page with a local variable'
# },
# )
# Helpers
# Methods defined in the helpers block are available in templates
# https://middlemanapp.com/basics/helper-methods/
# helpers do
# def some_helper
# 'Helping'
# end
# end
# Build-specific configuration
# https://middlemanapp.com/advanced/configuration/#environment-specific-settings
configure :build do
activate :minify_css
activate :minify_javascript
activate :minify_html
activate :gzip
activate :asset_hash
end
| {
"content_hash": "6c39defe4c0b72af40c63e0079d3ec54",
"timestamp": "",
"source": "github",
"line_count": 50,
"max_line_length": 80,
"avg_line_length": 20.94,
"alnum_prop": 0.7039159503342884,
"repo_name": "czuger/d10_fight_system",
"id": "c891c628fe5780c6cf6d526e1967bd067b726c45",
"size": "1158",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "middleman/config.rb",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "CSS",
"bytes": "2081"
},
{
"name": "HTML",
"bytes": "8386"
},
{
"name": "JavaScript",
"bytes": "8212"
},
{
"name": "Ruby",
"bytes": "59887"
}
],
"symlink_target": ""
} |
c Do the type of fitting as in fitz3.f but only at the given
c redshift, and return the coeffs of the best fit, for a
c list of objects/1d-spectra
c rework fittemplates to fit each spectrum w/a series of
c a single template broadened by various vel dispersions.
c fitdisp2 is like fitdisp but also does Monte Carlo realizations
c of the spectrum and refitting to get a hopefully more
c believable error bar.
program fitdisp2
include 'specarray.h'
c read spectrum names from a file?
parameter (IFFILE=1)
c plot intermediate steps?
parameter (IFPLOTALL=0)
c plot initial spectrum (first step)?
parameter (IFPLOTFIRST=1)
c polynomial or median continuum subtraction?
parameter(IFPOLYSUB=1)
c do cont sub/dev before blanking sky?
parameter(IFSUBFIRST=0)
c debug level
parameter(IFDEBUG=1)
c size of gaussian kernel for the broadening convolution
parameter(NGAUSMAX=401)
c number of broadenings
parameter(NBROADMAX=201)
c to conserve memory, don't allow 25 templates, just 5
c replace NMAXVECTS with NMAXTEMPL
c parameter(NMAXTEMPL=5)
c max number of monte carlo realizations
parameter (NMONTEMAX=201)
common /galvectors/ koffset,z,nvmax,nwlmax,ifitvect(NMAXVECTS),
$ evects(NMAXVECTS,NLOGWMAX)
c real broadvects(NBROADMAX,NMAXVECTS,NLOGWMAX)
real broadvects(NMAXVECTS,NBROADMAX,NLOGWMAX)
real spec1(NWAVEMAX),espec1(NWAVEMAX)
real spec2(NLOGWMAX),espec2(NLOGWMAX)
real spec3(NLOGWMAX),espec3(NLOGWMAX)
real specratio(NLOGWMAX),specratiofit(NLOGWMAX)
real tmpwl(NLOGWMAX),tmpspecfit(NLOGWMAX)
real tmpspec(NLOGWMAX),wavelog(NLOGWMAX),wavelin(NWAVEMAX)
real gauskern(NGAUSMAX),tmpxkern(NGAUSMAX)
real waverest1(NLOGWMAX)
real wrestlin(NWAVEMAX),tmpspec2(NWAVEMAX)
integer ifit(NLOGWMAX)
real wfit(NLOGWMAX),sfit(NLOGWMAX),efit(NLOGWMAX)
real rifit(NLOGWMAX),fitvect(NLOGWMAX)
real smcfit(NLOGWMAX)
real dispmonte(NMONTEMAX)
real disparray(NBROADMAX),chidisp(NBROADMAX),rchidisp(NBROADMAX)
character sname*160,esname*160,vname*160,oname*160
character answ*3,tlabel*160,xlabel*160
character namelabel*160,outfilename*80
character fsname*160,fename*160,fzname*160,fobjname*160
c integer isize(7)
c character charobjno*60
c stuff for svdfit. note these are dimensioned to nmaxvects
c but I also use them when fitting stars so they should really
c be the bigger of (nmaxvects,nstarvects)
real uu(NLOGWMAX,NMAXVECTS)
real vv(NMAXVECTS,NMAXVECTS),ww(NMAXVECTS)
real acoeff(NMAXVECTS),evals(NMAXVECTS)
c real chifit(NMAXSTEP),rchifit(NMAXSTEP)
c real chifitstar(NMAXSTEP),rchifitstar(NMAXSTEP)
real dacoeff(NMAXVECTS),covar(NMAXVECTS,NMAXVECTS)
c real doffit(NMAXSTEP),doffitstar(NMAXSTEP)
external galfuncs
c external gasdev
include 'pcredshift.h'
root2pi = sqrt(2.0*3.141593)
root2 = sqrt(2.0)
idum=-1
nmonte=50
call pgbeg(0,'?',1,1)
call pgscf(2)
call pgsch(1.3)
nxplot=1
nyplot=1
write(*,'("Full continuum fit subtraction [1,y-yes]? ",$)')
read(*,'(a1)') answ
if (answ(1:1) .eq. '0' .or. answ(1:1) .eq. 'n' .or.
$ answ(1:1) .eq. 'N') then
ifcontsub = 0
else
ifcontsub = 1
end if
100 write(*,
$ '("Diameter for median smoothing, pixels [0,1=none]: ",$)')
read(*,'(a3)') answ
if (answ(1:3) .eq. ' ') then
ndiamed = 0
else
read(answ,*,err=100) ndiamed
end if
nvmax=NMAXVECTS
nwlmax=NLOGWMAX
nvbroadmax=NBROADMAX
nvbroad = 121
dispstep = 5.
write (*,'("Read pre-smoothed template set? 0/1 ",$)')
read (*,*) ifpresmooth
c open file and read eigenvectors. nv is returned as
c the # of vectors to use, nw is the actual # of wavelength pixels
c so now evects is the unbroadened templates
call readevects(evects,nvmax,nwlmax,waverest1,nv,nw)
nwlog = nw
dwrlog = (waverest1(nw) - waverest1(1)) / (nwlog-1.)
w0rlog = waverest1(1) - dwrlog
do i=1,nwlog
wrestlin(i) = 10**waverest1(i)
end do
c figure out w0 and dw for the eigenvectors. This is in log w
c There is no check yet to make sure it's evenly spaced.
dwrlog = (waverest1(nw) - waverest1(1)) / (nwlog-1.)
w0rlog = waverest1(1) - dwrlog
do i=1,nwlog
wrestlin(i) = 10**waverest1(i)
end do
write(*,*) "w0rlog, dwrlog = ", w0rlog,dwrlog
200 continue
if (IFFILE .eq. 1) then
write(*,'("File with spectrum names: ",$)')
read(*,'(a)') fsname
open(10,file=fsname,status='old',err=200)
202 write(*,'("File with actual redshifts: ",$)')
read(*,'(a)') fzname
open(12,file=fzname,status='old',err=202)
ifzfile=1
end if
210 write(*,'("fit output file [fitdisp1.out]: ",$)')
read(*,'(a)') oname
if (oname(1:3) .eq. ' ') oname='fitdisp1.out'
open(8,file=oname,status='unknown',err=210)
215 write(*,'("disp output file [fitdisp2.out]: ",$)')
read(*,'(a)') oname
if (oname(1:3) .eq. ' ') oname='fitdisp2.out'
open(9,file=oname,status='unknown',err=210)
c 217 write(*,'("chisq array output file [fitdisp3.out]: ",$)')
c read(*,'(a)') oname
c if (oname(1:3) .eq. ' ') oname='fitdisp3.out'
c open(7,file=oname,status='unknown',err=210)
c old - as an expedient, construct a set of smoothed vectors
c by smoothing the first one, i.e. we are discarding all but
c the first vector
c smooth each of the input vectors in evects to fill up
c broadvects, which has dimensions nvbroadmax,nvmax,nwlmax
c or nvmax,nvbroadmax,nwlmax
c smoothing by a dv const. w/wavelength is also smoothing by
c a d(log wavelength) const w/wave
c reading presmoothed does not yet work in the more-than-one-template
c version
if (ifpresmooth .eq. 1) go to 180
c dispstep = 10.
disparray(1) = 0.0
do k=2,nvbroad
disparray(k) = disparray(1) + (k-1) * dispstep
end do
c loop over the nv templates
do itempl=1,nv
call pgenv(waverest1(1),waverest1(nwlog),0.,1000.,0,1)
write(tlabel,'("template ",i3)') itempl
call pglabel("log wavelength","flux",tlabel)
do j=1,nwlog
tmpspec(j) = evects(itempl,j)
end do
call pgline(nwlog,waverest1,tmpspec)
c copy the first set in unchanged
do j=1,nwlog
broadvects(itempl,1,j) = evects(itempl,j)
end do
c loop over broadenings
do k = 2,nvbroad
displogw = disparray(k) / 3.0e5 / 2.3026
displogpix = displogw/dwrlog
displogpixsq = displogpix**2
c should really move the entire gaussian convol to a subroutine
iradkern = int(4.0*displogpix)
iradkern = min(iradkern,(NGAUSMAX-1)/2)
ngauskern = 2*iradkern+1
ikernctr = 1+iradkern
gkernsum = 0.0
do j=1,ngauskern
c This was a direct gaussian, while we really ought to be
c using error functions. Not sure this really makes a
c signif difference.
gauskern(j) = 1./root2pi/displogpix *
$ exp(-(j-ikernctr)**2/2.0/displogpixsq)
c erf(x) is integral exp(-x^2)
c if (j .ne. ikernctr) then
c gauskern(j) = 1./root2pi/displogpix *
c $ abs( erf((j+0.5-ikernctr)/displogpix/root2) -
c $ erf((j-0.5-ikernctr)/displogpix/root2) )
c else
c gauskern(j) = 2. * 1./root2pi/displogpix *
c $ abs( erf(-(j+0.5-ikernctr)/displogpix/root2) )
c end if
c this ought to come out near 1
gkernsum = gkernsum + gauskern(j)
end do
c if (IFDEBUG .ge. 1) then
c ifplotkern=1
c if (ifplotkern .ge. 1 .and. mod(k,20) .eq. 1) then
cc write(*,*) k,disparray(k),displogpix,gkernsum
c do j=1,ngauskern
c tmpxkern(j) = (j-ikernctr)*displogpix
c end do
c call pgenv(tmpxkern(1),tmpxkern(ngauskern),
c $ 0.,gauskern(ikernctr)*1.05,0,1)
c call pgline(ngauskern,tmpxkern,gauskern)
c write(tlabel,'("disp ",f7.1," displogpix ",f5.2)')
c $ disparray(k),displogpix
c call pglabel("log disp","kernel",tlabel)
c end if
do j=1,ngauskern
gauskern(j) = gauskern(j) /gkernsum
end do
c loop over wavelength, filling into the broadvects array
do i=1,nwlog
jmin = max(1,i-iradkern)
jmax = min(nwlog,i+iradkern)
c evects(k,i) = 0.0
broadvects(itempl,k,i) = 0.0
do j=jmin,jmax
c evects(k,i) = evects(k,i) +
c $ evects(1,j)*gauskern(ikernctr+j-i)
broadvects(itempl,k,i) = broadvects(itempl,k,i) +
$ evects(itempl,j)*gauskern(ikernctr+j-i)
end do
end do
if (IFDEBUG .ge. 2 .and. mod(k,5) .eq. 0) then
call pgenv(waverest1(1),waverest1(nwlog),0.,1000.,0,1)
do j=1,nwlog
tmpspec(j) = broadvects(itempl,k,j)
end do
call pgline(nwlog,waverest1,tmpspec)
end if
end do
c end the looping over templates
end do
c write out smoothed vectors for next time
c this doesn't yet work in the multi-template version
open(4,file='smoothvects.out',status='unknown')
do j=1,nwlog
write(4,'(f8.6,$)') waverest1(j)
do itempl=1,nv
c write(4,'(f8.2,$)') evects(itempl,j)
write(4,'(f8.2,$)') broadvects(itempl,1,j)
end do
write(4,*)
end do
180 continue
c continuum subtract the template vectors
c dummy array of errors
do i=1,nwlog
tmpspec2(i) = 1.0
end do
c loop over templates
do itempl=1,nv
do k=1,nvbroad
do j=1,nwlog
c tmpspec(j) = evects(k,j)
tmpspec(j) = broadvects(itempl,k,j)
end do
if (ifcontsub .ne. 0) then
contwrad = 100.*dwrlog
call contdivpctile(tmpspec,nwlog,tmpspec2,dwrlog,contwrad)
c if(IFPOLYSUB .ne. 0) then
cc call contsubpoly(wavelog,spec3,espec3,nwlog,15)
cc call contsubleg(wavelog,spec3,espec3,nwlog,15)
c call contsubleg(waverest1,tmpspec,tmpspec2,
c $ nwlog,15)
c else
c contwrad = 100.*dwrlog
c call contsubmed(tmpspec,nwlog,dwrlog,contwrad)
c end if
else
c call contsubconst(evects(k,1),nwlog)
call contsubconst(broadvects(itempl,k,1),nwlog)
end if
do j=1,nwlog
c evects(k,j) = tmpspec(j)
broadvects(itempl,k,j) = tmpspec(j)
end do
end do
c end looping over templates
end do
c in the multi-template version, this only writes the
c unbroadened (but continuum divided) vectors
open(4,file='csubvects.out',status='unknown')
do j=1,nwlog
write(4,'(f8.6,$)') waverest1(j)
do itempl=1,nv
write(4,'(f8.2,$)') broadvects(itempl,1,j)
end do
write(4,*)
end do
c plot some of the template vectors
call pgsubp(2,2)
do itempl=1,nv
do k=1,nvbroad,20
do j=1,nwlog
c tmpspec(j) = evects(k,j)
tmpspec(j) = broadvects(itempl,k,j)
end do
call showspec(nwlog,waverest1,tmpspec)
write(tlabel,'(2(a,1x,i3))') "template",itempl," broadening ",k
call pglabel("log wavelength"," ",tlabel)
end do
end do
cc advance to next full page
c ipage = mod(4-nv,4)
c do i=1,ipage
c call pgpage
c end do
call pgsubp(2,2)
call pgsubp(nxplot,nyplot)
c Here we start looping over objects
220 continue
c open files and read spec and error, with wavelength calib.
if (IFFILE .eq. 1) then
read(10,'(a)',err=666,end=666) sname
read(12,*,err=666,end=666) zreal
end if
c label for tops of plot (objno would be better)
c use up to last 40 chars of spectrum name
iend = index(sname,' ')-1
istart = max(1,iend-39)
write(namelabel,'(a)') sname(istart:iend)
c this linearizes the spectrum which may be less than ideal
c call get1ddeepspec(sname,nwspec,spec1,espec1,w0sp,dwsp,ier)
call get1dcoaddspec(sname,nwspec,spec1,espec1,w0sp,dwsp,ier)
if (ier .ne. 0) then
c write something to the output files?
write(*,'("couldnt open ",a)') sname
write(8,'("couldnt open ",a)') sname
write(9,'("couldnt open ",a)') sname
go to 220
end if
write(*,*) "nwspec, w0sp, dwsp = ", nwspec,w0sp,dwsp
c zero out anything beyond the actual spectrum
do i=nwspec+1,NWAVEMAX
spec1(i) = 0.0
espec1(i) = 0.0
end do
c try to clean out bad values
c feb 11 2008 - added a check for very small error values, which
c crept in in a test spectrum
c also add a computation of median SNR
badmax = 10000.
errmin = 1.0e-5
nwspecgood=0
do i=1,nwspec
if(spec1(i) .lt. bad .or. spec1(i) .gt. badmax .or.
$ espec1(i) .lt. errmin) then
spec1(i) = badset
c if(espec1(i) .lt. bad .or. espec1(i) .gt. badmax)
espec1(i) = badset
else
nwspecgood=nwspecgood+1
tmpspec2(nwspecgood) = spec1(i)/espec1(i)
end if
end do
c find the mean/median SNR
snrmean = 0.0
do i=1,nwspecgood
snrmean = snrmean + tmpspec2(i)
end do
snrmean = snrmean / nwspecgood
imed = nint(nwspecgood/2.0)
snrmed = select(imed,nwspecgood,tmpspec2)
write(*,'(" SNR mean, median: ",f7.3,1x,f7.3)') snrmean,snrmed
do i=1,nwspec
wavelin(i) = w0sp+i*dwsp
c this was wrong or not useful
c wavelog(i) = log10(wavelin(i))
end do
if (IFPLOTALL .ne. 0 .or. IFPLOTFIRST .ne. 0) then
call showspec(nwspec,wavelin,spec1)
call pgqci(indexc)
call pgsci(3)
call pgline(nwspec,wavelin,espec1)
call pgsci(indexc)
write (tlabel,'(a,", z= ",f8.4)') "spectrum and error",zreal
call pglabel("wavelength","counts",tlabel)
end if
if (ndiamed .gt. 1) then
call medsmooth(nwspec,spec1,ndiamed,spec2)
else
do i=1,nwspec
spec2(i) = spec1(i)
end do
end if
do i=1,nwspec
espec2(i) = espec1(i)
end do
if (IFPLOTALL .ne. 0 .or. IFPLOTFIRST .ne. 0
$ .and. ndiamed .gt. 1) then
call showspec(nwspec,wavelin,spec2)
call pglabel("wavelength","counts","median smoothed")
call pgqci(indexc)
call pgsci(3)
call pgline(nwspec,wavelin,espec2)
call pgsci(indexc)
end if
nwmedsmgood=0
do i=1,nwspec
if (spec2(i) .gt. bad) nwmedsmgood=nwmedsmgood+1
end do
c do continuum subtraction before blanking, which at the
c moment means doing it before log rebinning? Or not
if (ifcontsub .ne. 0 .and. IFSUBFIRST .eq. 1) then
c save the pre-subtractiion spectrum. This is before log rebinning
c and blanking. If we do this then we have to log rebin
c tmpspec2 into specratio to be consistent with later.
do i=1,nwspec
tmpspec2(i) = spec2(i)
end do
call logrebin(tmpspec2,nwspec,w0sp,dwsp,nwlog,w0rlog,dwrlog,
$ specratio)
c note dwlog not defined yet, could use dwrlog
c contwrad = 100.*dwlog
c call contdivpctile(spec3,nwlog,espec3,dwlog,contwrad)
contwrad = 150.*dwsp
call contdivpctile(spec2,nwspec,espec2,dwsp,contwrad)
c if(IFPOLYSUB .ne. 0) then
cc call contsubpoly(wavelog,spec3,espec3,nwlog,15)
cc call contsubleg(wavelog,spec3,espec3,nwlog,15)
c call contsubleg(wavelog(imin),spec3(imin),espec3(imin),
c $ imax-imin+1,15)
c else
c contwrad = 100.*dwlog
c call contsubmed(spec3,nwlog,dwlog,contwrad)
c end if
else
c call contsubconst(spec3,nwlog)
call contsubconst(spec2,nwsp)
end if
c easier to deal with cont sub, etc if we blank later, after
c cont subtraction etc.
c call blankoutsky2(spec1,1,1,nwspec,wavelin)
c call selectabsregions(spec1,1,1,nwspec,wavelin,zreal)
call blankoutsky2(spec2,1,1,nwspec,wavelin)
c Blank out emission lines too. This needs to know
c z so we can blank in the restframe
call blankoutlines(spec2,1,1,nwspec,wavelin,zreal)
c call selectabsregions(spec2,1,1,nwspec,wavelin,zreal,0)
if (IFPLOTALL .ne. 0) then
call showspec(nwspec,wavelin,spec2)
call pglabel("wavelength","counts","sky blanked")
end if
c change wavelength scale to zero redshift
w0sp = w0sp / (1. + zreal)
dwsp = dwsp / (1. + zreal)
cc Figure out the ref wavelength for the log rebinning so that
cc it falls on the wl scale of the eigenvectors.
c tmp = log10(w0sp)
c itmp = int( (tmp-w0rlog)/dwrlog )
c w0log = w0rlog + dwrlog*itmp
cc k0offset is the offset in integer index of log wavelength
cc from the beginning of the eigenvectors to the spectrum to be fit.
c k0offset = itmp
c dwlog = dwrlog
c Rather than doing the above, make it simple and rebin the
c spectrum onto the exact wavelength scale of the eigenvectors
w0log = w0rlog
dwlog = dwrlog
itmp = 0
k0offset = 0
write(*,*) "w0log, dwlog = ", w0log,dwlog
call logrebinerr(spec2,espec2,nwspec,w0sp,dwsp,nwlog,w0log,dwlog,
$ spec3,espec3)
do i=1,nwlog
wavelog(i) = w0log + i*dwlog
end do
if (IFPLOTALL .ne. 0) then
call showspec(nwlog,wavelog,spec3)
call pglabel("log rest wavelength","counts","log rebinned")
call pgqci(indexc)
call pgsci(3)
call pgline(nwlog,wavelog,espec3)
call pgsci(indexc)
end if
nwlogrebgood=0
do i=1,nwlog
if (spec3(i) .gt. bad) nwlogrebgood=nwlogrebgood+1
end do
call findends(nwlog,spec3,imin,imax)
call cleanends(nwlog,spec3,imin,imax)
write(*,*) "imin, imax = ", imin,imax
c cont sub if doing it later
if (ifcontsub .ne. 0 .and. IFSUBFIRST .eq. 0) then
c save the pre-division spectrum. This is after log rebinning
do i=1,nwlog
specratio(i) = spec3(i)
end do
c contdivpctile divides or subtracts by the 90th %ile of pixels
c in some running bin. Currently set up to have it divide.
contwrad = 150.*dwlog
call contdivpctile(spec3,nwlog,espec3,dwlog,contwrad)
c contwrad = 100.*dwsp
c call contdivpctile(spec2,nwspec,espec2,dwsp,contwrad)
c if(IFPOLYSUB .ne. 0) then
cc call contsubpoly(wavelog,spec3,espec3,nwlog,15)
cc call contsubleg(wavelog,spec3,espec3,nwlog,15)
c call contsubleg(wavelog(imin),spec3(imin),espec3(imin),
c $ imax-imin+1,15)
c else
c contwrad = 100.*dwlog
c call contsubmed(spec3,nwlog,dwlog,contwrad)
c end if
else
call contsubconst(spec3,nwlog)
c call contsubconst(spec2,nwsp)
end if
c Set specratio to be the ratio of pre-continuum removal to after.
do i=1,nwlog
specratio(i) = specratio(i)/spec3(i)
end do
c write(*,*) 'got here'
if (IFPLOTALL .ne. 0) then
call showspec(nwlog,wavelog,spec3)
call pgqci(indexc)
call pgsci(3)
call pgline(nwlog,wavelog,espec3)
call pgsci(indexc)
call pglabel("log rest wavelength","counts",
$ "continuum subtracted")
end if
nwcsubgood=0
do i=1,nwlog
if (spec3(i) .gt. bad) nwcsubgood=nwcsubgood+1
end do
c select regions with abs lines, in log wavelength.
c at this point the spectrum is already shifted to rest,
c so send in z=0.0
c call selectabsregions(spec3,1,1,nwlog,wavelog,0.0,1)
c copy wave and spec to temp array, cleaning out bad points
npfit=0
do i=1,nwlog
if(spec3(i) .gt. bad .and. espec3(i) .gt. bad) then
npfit=npfit+1
ifit(npfit) = i
rifit(npfit) = real(i)
wfit(npfit) = w0log + i*dwlog
sfit(npfit) = spec3(i)
efit(npfit) = espec3(i)
c This is for plotting later
specratiofit(npfit) = specratio(i)
end if
end do
write (*,*) npfit, " points to fit"
write (*,'("npts by stage: ",6(3x,i6))') nwspec,nwspecgood,
$ nwmedsmgood,nwlog,nwlogrebgood,nwcsubgood
if (npfit .le. 0) then
write(*,*) "Error! No points to fit!"
c do i=1,nfind
c zestarr(i) = 0.0
c end do
c go to 400
npfittmp = 0
write(*,'(a,3(2x,f8.2))') "best disp and err range: ",
$ -999,-10000,10000
write(9,'(a40,3(2x,f8.2))') namelabel,
$ -999,-10000,10000
write(8,'(1pe12.5,$)') -999
do j=1,nv
write(8,'(2(1x,1pe10.3),$)') -999.,10000
end do
write(8,'(2x,f8.5)') zreal
go to 220
end if
c don't really need to show this if we later overplot best fit
c call showspec(npfit,wfit,sfit)
c call pgqci(indexc)
c call pgsci(3)
c call pgline(npfit,wfit,efit)
c call pgsci(indexc)
c call pglabel("log rest wavelength","counts",
c $ "spec to fit, "//namelabel)
c The spectrum-to-fit may have gaps, while the templates should
c not. In fitz3, rather than re-align the templates with the
c good points in the spectrum at every lag, I used an indirect
c reference to the data - the "x" over which the fit is made
c is rifit, which is just a real version of the array index;
c as opposed to using wfit, which is the actual wavelength.
c Then galfuncs uses this array index to find the template values.
c For the fittemplates application, since I'm only using a
c zero lag, this isn't really necessary, but I'll stick with it.
c At least this means we don't have to do any complicated
c dorking around with indexes for min and max points to fit.
iminfit = 1
npfittmp = npfit
c koffset is the offset in index between spectrum-to-fit
c and templates, which is passed through to galfuncs in a common block.
c Since I put the spectrum onto the log scale of the evects (zero lag),
c it is zero here.
koffset = 0
c call bigsvdfit(rifit(iminfit),sfit(iminfit),efit(iminfit),
c $ npfittmp,acoeff,nv,uu,vv,ww,nwlmax,nvmax,chisq1,galfuncs)
cc chifit(kindex) = chisq1
cc get error bars on coefficients
c call svdvar(vv,nv,nvmax,ww,covar,nvmax)
c do i=1,nv
c dacoeff(i) = sqrt(covar(i,i))
c end do
c for fitdisp, I really just want to fit a single template at a time
c and all I really need is one coefficient. In principle there should
c not even be a DC offset since both are continuum subtracted.
c control which vector to fit by passing in an integer array
c through galvectors common block to galfuncs; this doesn't fit
c the dc offset
c for multi-template fitdisp, loop over broadening;
c at each value, copy the appropriately broadened templates
c into the evects array, which is what galfuncs actually has
c access to through the common block. There is probably
c a more elegant and faster way to do this.
c if we are allowing the first fit coeff to be the DC level
nvfit = nv+1
c nvfit = nv
do i=1,nvfit
ifitvect(i) = 1
end do
do i=nvfit+1,nvmax
ifitvect(i) = 0
end do
do k=1,nvbroad
c do i=1,nv
c ifitvect(i) = 0
c end do
c ifitvect(k) = 1
do itempl=1,nv
do j=1,nwlog
evects(itempl,j) = broadvects(itempl,k,j)
end do
end do
call bigsvdfit(rifit(iminfit),sfit(iminfit),efit(iminfit),
c $ npfittmp,acoeff,nvmax,uu,vv,ww,nwlmax,nvmax,chisq1,galfuncs)
$ npfittmp,acoeff,nvfit,uu,vv,ww,nwlmax,nvmax,chisq1,galfuncs)
c chifit(kindex) = chisq1
chidisp(k) = chisq1
c get error bars on coefficients - no real need for error bars here
c call svdvar(vv,nvmax,nvmax,ww,covar,nvmax)
c call svdvar(vv,nv,nvmax,ww,covar,nvmax)
c do i=1,nv
c dacoeff(i) = sqrt(covar(i,i))
c end do
c end looping over broadening
end do
write(*,*) "used ",npfittmp," points"
rchimin = 1.e10
rchimax = -1.e10
do k=1,nvbroad
rchidisp(k) = chidisp(k) / npfittmp
if (rchidisp(k) .lt. rchimin) kmin = k
rchimin = min(rchimin,rchidisp(k))
c don't count ridiculous values
if (rchidisp(k) .lt. 50.) rchimax = max(rchimax,rchidisp(k))
c write(9,'(1pe13.6,1x,$)') rchidisp(k)
c write(*,'(f4,2x,1pe13.6,)') disparray(k),rchidisp(k)
end do
c write(9,*)
write(*,*) "Best chi-sq at ",kmin,disparray(kmin)
c now we have an array of chi-sq vs dispersion
c need to find the minimum and error range
c look for a delta-total-chisq of 1, scaled by whatever the
c min rchisq was, as if we scaled up the error bars to get
c rchisq to come out to 1. If rchisq was <1, don't scale down.
c The errors this way don't seem to be big enough.
drchisq = 1.0/npfittmp * max(1.0, rchidisp(kmin))
call findminerr2(nvbroad,disparray,rchidisp,drchisq,
$ dispmin,disperrlo,disperrhi)
c plot the array and min
call pgenv(disparray(1),disparray(nvbroad),rchimin,rchimax,0,1)
call pglabel("velocity dispersion","reduced chi-squared",
$ namelabel)
call pgpoint(nvbroad,disparray,rchidisp,17)
call pgqci(indexc)
call pgsci(2)
call pgpt1(disparray(kmin),rchidisp(kmin),12)
c yplot = rchidisp(kmin)*1.1
yplot = rchimin + 0.3*(rchimax-rchimin)
call pgpt1(dispmin,yplot,17)
call pgerr1(1,dispmin,yplot,disperrhi-dispmin,1.0)
call pgerr1(3,dispmin,yplot,-disperrlo+dispmin,1.0)
call pgsci(indexc)
write(*,'(a,3(2x,f8.2))') "best disp and err range: ",
$ dispmin,disperrlo,disperrhi
c write(9,'(a40,3(2x,f8.2))') namelabel,
c $ dispmin,disperrlo,disperrhi
c To plot best fit vector:
c I think I need to figure out the min of rchi, go to that value
c of vel (really the proper index k) and rerun bigsvdfit to get acoeff
c right.
c do i=1,nvmax
c ifitvect(i) = 0
c end do
c ifitvect(kmin) = 1
do itempl=1,nv
do j=1,nwlog
evects(itempl,j) = broadvects(itempl,kmin,j)
end do
end do
call bigsvdfit(rifit(iminfit),sfit(iminfit),efit(iminfit),
c $ npfittmp,acoeff,nvmax,uu,vv,ww,nwlmax,nvmax,chisq1,galfuncs)
$ npfittmp,acoeff,nvfit,uu,vv,ww,nwlmax,nvmax,chisq1,galfuncs)
c get error bars on coeffs of the best fit
c write out best coeffs
call svdvar(vv,nvfit,nvmax,ww,covar,nvmax)
write(*,'(a,$)') "best-fit coeffs:"
do i=1,nvfit
dacoeff(i) = sqrt(covar(i,i))
write(*,'(2x,1pe10.3," +-",1pe10.3,$)') acoeff(i),dacoeff(i)
end do
write(*,*)
c compute best fit spectrum
c should this be npfittmp???
do i=1,npfit
c call galfuncs(rifit(i),evals,nvmax)
call galfuncs(rifit(i),evals,nvfit)
fitvect(i) = 0.0
do j=1,nvfit
fitvect(i) = fitvect(i) + acoeff(j)*evals(j)
end do
end do
write(8,'(1pe12.5,$)') chisq1/real(npfittmp)
do j=1,nvfit
write(8,'(2(1x,1pe10.3),$)') acoeff(j),dacoeff(j)
end do
c write(2,'(2x,f8.5,2x,a100)') zreal,sname
write(8,'(2x,f8.5)') zreal
c make a lightly smoothed version of the data, for plotting
c make the smoothing constant in dw, so if we use a more finely
c sampled template we smooth more
iwid = int(2.e-4/dwlog)
c smooth sfit and store in spec2
call medsmooth(npfit,sfit,iwid,spec2)
c call showspec(npfit,wfit,sfit)
call showspec(npfit,wfit,spec2)
call pglabel("log rest wavelength","counts"," ")
call pgmtxt('T',2.5,0.0,0.0,namelabel)
write(tlabel,'(a,", z= ",f8.4)') "spectrum and best fit",zreal
c call pgmtxt('T',1.5,0.0,0.0,"spectrum and best fits")
call pgmtxt('T',1.5,0.0,0.0,tlabel)
call pgqci(indexc)
call pgsci(2)
call pgline(npfit,wfit,fitvect)
c write(tlabel,'(a,f7.4)') "chi-sq, z=",zmin
c call pgmtxt('T',2.5,1.0,1.0,tlabel)
call pgsci(indexc)
c overplot fitted vectors
c Try plotting with the continuum multiplied back in.
c We could also try plotting on a linear wavelength scale
do i=1,npfit
tmpspec(i) = sfit(i) * specratiofit(i)
tmpwl(i) = 10**wfit(i)
tmpspecfit(i) = fitvect(i) * specratiofit(i)
end do
c Would like to make a vector of the best-fit model that showed
c model through the blanked-out wavelengths
c do i=1,nwlog
c tmpwl2(i) = 10**wavelog(i)
c Can't quite do this since I would have to pass wavelengths into
c galfuncs somehow to get the evaluated model
c tmpspecfit2(i) =
c end do
c smooth, reuse spec2 for temp storage of the smooth vector to plot
call medsmooth(npfit,tmpspec,iwid,spec2)
c plot in log wavelength
call showspec(npfit,wfit,spec2)
call pglabel("log rest wavelength","normalized counts"," ")
call pgmtxt('T',2.5,0.0,0.0,namelabel)
write(tlabel,'(a,", z= ",f8.4)') "spectrum and best fit",zreal
c call pgmtxt('T',1.5,0.0,0.0,"spectrum and best fits")
call pgmtxt('T',1.5,0.0,0.0,tlabel)
call pgqci(indexc)
call pgsci(2)
call pgline(npfit,wfit,tmpspecfit)
c write(tlabel,'(a,f7.4)') "chi-sq, z=",zmin
c call pgmtxt('T',2.5,1.0,1.0,tlabel)
call pgsci(indexc)
c do the overplot in linear wavelength
c Here I could plot the model even where the data was blanked out
c but constructing it is extra work and not done yet.
call showspec(npfit,tmpwl,spec2)
call pglabel("rest wavelength","counts"," ")
call pgmtxt('T',2.5,0.0,0.0,namelabel)
write(tlabel,'(a,", z= ",f8.4)') "spectrum and best fit",zreal
c call pgmtxt('T',1.5,0.0,0.0,"spectrum and best fits")
call pgmtxt('T',1.5,0.0,0.0,tlabel)
call pgqci(indexc)
call pgsci(2)
c call pgline(npfit,tmpwl,tmpspecfit)
call pgline(npfit,tmpwl,tmpspecfit)
c write(tlabel,'(a,f7.4)') "chi-sq, z=",zmin
c call pgmtxt('T',2.5,1.0,1.0,tlabel)
call pgsci(indexc)
c Try to do a Monte Carlo to find the errors on dispersion.
c Do this by taking the best fit model, rewiggling each point
c by the error bar to make a new spectrum, and refitting that.
c nmonte = 50
do ii=1,nmonte
do i=1,npfit
smcfit(i) = fitvect(i) + gasdev(idum)*efit(i)
end do
do k=1,nvbroad
do itempl=1,nv
do j=1,nwlog
evects(itempl,j) = broadvects(itempl,k,j)
end do
end do
call bigsvdfit(rifit(iminfit),smcfit(iminfit),efit(iminfit),
c $ npfittmp,acoeff,nvmax,uu,vv,ww,nwlmax,nvmax,chisq1,galfuncs)
$ npfittmp,acoeff,nvfit,uu,vv,ww,nwlmax,nvmax,chisq1,galfuncs)
c chifit(kindex) = chisq1
chidisp(k) = chisq1
end do
rchimin = 1.e10
rchimax = -1.e10
do k=1,nvbroad
rchidisp(k) = chidisp(k) / npfittmp
if (rchidisp(k) .lt. rchimin) kmin = k
rchimin = min(rchimin,rchidisp(k))
c don't count ridiculous values
if (rchidisp(k) .lt. 50.) rchimax = max(rchimax,rchidisp(k))
c write(9,'(1pe13.6,1x,$)') rchidisp(k)
c write(*,'(f4,2x,1pe13.6,)') disparray(k),rchidisp(k)
end do
dispmonte(ii) = disparray(kmin)
end do
sumd = 0.0
sumdsq = 0.0
do ii=1,nmonte
sumd = sumd + dispmonte(ii)
sumdsq = sumdsq + dispmonte(ii)**2
end do
avgdispmonte = sumd/nmonte
rmsdispmonte = sqrt(sumdsq/nmonte - avgdispmonte**2)
write(9,'(a40,6(2x,f8.2))') namelabel,
$ dispmin,disperrlo,disperrhi,avgdispmonte,rmsdispmonte,snrmed
write(*,'(a40,6(2x,f8.2))') namelabel,
$ dispmin,disperrlo,disperrhi,avgdispmonte,rmsdispmonte,snrmed
c necessary?
c call pgsubp(nxplot,nyplot)
go to 220
666 continue
close(8)
close(9)
call pgend()
end
cccccccccccccccccccc
cccccccccccccccccccc
c galfuncs returns the values of the nv eigenvectors
c evaluated at position xfit, in the array evals
c if svdfit is called with argument wfit, xfit is the
c log wavelength.
c if svdfit is called with argument rifit, xfit is the
c index in the log wavelength array, as a real.
c
c if we are allowing a DC component the first func is a constant
subroutine galfuncs(xfit,evals,nterms)
include 'specarray.h'
real evals(nterms)
common /galvectors/ koffset,z,nvmax,nwlmax,ifitvect(NMAXVECTS),
$ evects(NMAXVECTS,NLOGWMAX)
c find the index corresponding to the position xfit
c and retrieve the eigenvector values
c this is for use with rifit
ispec = nint(xfit)
jvect = ispec-koffset
if(jvect .ge. 1 .and. jvect .le. nwlmax) then
evals(1) = 1.0
do i=2,nterms
c allow ifitvect to control what vectors are actually fit
if (ifitvect(i) .ne. 0) then
evals(i) = evects(i-1,jvect)
else
evals(i) = 0.0
end if
end do
else
do i=1,nterms
evals(i) = 0.0
end do
end if
return
end
c given an array of y and a delta-y, find the x where y is minimum
c and x error range
subroutine findminerr(n,x,y,deltay,xmin,xerrlo,xerrhi)
real x(n),y(n)
c first just find the min point
ymin = 1.0e20
do i=1,n
if (y(i) .lt. ymin) then
ymin = y(i)
xmin = x(i)
imin = i
end if
end do
ythresh = ymin + deltay
c now step away to find where we go above ythresh.
c This assumes that the array is fairly smooth. could
c also look for the min and max points above ythresh
c which is what findminerr2 does
ilo=imin-1
2010 continue
if (y(ilo) .lt. ythresh .and. ilo .gt. 1) then
ilo = ilo-1
go to 2010
end if
if (ilo .eq. 1) then
xerrlo = x(1)
else
frac = (ythresh-y(ilo)) / (y(ilo+1)-y(ilo))
xerrlo = frac*x(ilo+1) + (1.-frac)*x(ilo)
end if
c now step up
ihi = imin+1
2020 continue
if (y(ihi) .lt. ythresh .and. ihi .lt. n) then
ihi = ihi+1
go to 2020
end if
if (ihi .eq. n) then
xerrhi = x(n)
else
frac = (ythresh-y(ihi-1)) / (y(ihi)-y(ihi-1))
xerrhi = frac*x(ihi) + (1.-frac)*x(ihi-1)
end if
c it would be better to do the whole thing on a splined version
return
end
c given an array of y and a delta-y, find the x where y is minimum
c and the x error range
subroutine findminerr2(n,x,y,deltay,xmin,xerrlo,xerrhi)
real x(n),y(n)
c first just find the min point
ymin = 1.0e20
do i=1,n
if (y(i) .lt. ymin) then
ymin = y(i)
xmin = x(i)
imin = i
end if
end do
ythresh = ymin + deltay
c now step away to find where we go above ythresh.
c This assumes that the array is fairly smooth.
c in this version, we go all the way to the end of teh
c array, in case there are secondary minima.
c Start with the xerrlo and xerrhi being at least 1 grid
c point away (I didn''t bother interpolating)
xerrlo = x(imin-1)
do i=imin-1,1,-1
if (y(i) .lt. ythresh) xerrlo = x(i)
end do
xerrhi = x(imin+1)
do i=imin+1,n
if (y(i) .lt. ythresh) xerrhi = x(i)
end do
return
end
| {
"content_hash": "efd6c99cbdbc6f5bfc2d5bc47470ad9e",
"timestamp": "",
"source": "github",
"line_count": 1070,
"max_line_length": 73,
"avg_line_length": 33.73271028037383,
"alnum_prop": 0.6119022552224747,
"repo_name": "bjweiner/DEEP2-redshift",
"id": "2958d2b6a350c09bb8b16c1135d42a5cadfd55af",
"size": "36095",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "fitdisp2.f",
"mode": "33188",
"license": "mit",
"language": [],
"symlink_target": ""
} |
package org.diirt.graphene.profile.settings;
import java.awt.image.BufferedImage;
import java.lang.management.ManagementFactory;
/**
* A general purpose collection of messages to be saved to a
* .CSV output file for profiling.
* <p>
* Includes various properties,
* such as author and dataset comments,
* as well as hardware comments such as RAM.
*
* @author asbarber
*/
public class SaveSettings implements Settings{
/**
* Conversion from bytes to gigabytes:
* 2^30 BYTES per GB.
*/
private static final double BYTES_TO_GB = 1073741824; //2^30
//Member Fields
//--------------------------------------------------------------------------
private String datasetMessage = "",
saveMessage = "",
authorMessage = "";
private BufferedImage saveImage = null;
//--------------------------------------------------------------------------
//Setters
//--------------------------------------------------------------------------
/**
* Set the comment associated with the data set.
* This comment will be written to the CSV log file when saving the statistics.
* <p>
* This is appropriate for discussing the distribution of the data, dimensions of the data, etc.
*
* @param message comment about the data
*/
public void setDatasetMessage(String message){
this.datasetMessage = message;
}
/**
* Set the general comment associated with the profile.
* This comment will be written to the CSV log file when saving the statistics.
* <p>
* This is appropriate for discussing the parameters of the renderer, etc.
*
* @param message general comment about the profiling
*/
public void setSaveMessage(String message){
this.saveMessage = message;
}
/**
* Set the author associated with the profile.
* This comment will be written to the CSV log file when saving the statistics.
* <p>
* This is appropriate for stating the user who profiled.
* This can help provide understanding in different results due
* to computer hardware differences.
*
* @param author author for the profiling
*/
public void setAuthorMessage(String author){
this.authorMessage = author;
}
/**
* Sets the image to be saved.
* The image is one of the images rendered during profiling.
* This field is not written to the comments of the CSV log file.
* @param saveImage image of the graph to be saved
*/
public void setSaveImage(BufferedImage saveImage){
this.saveImage = saveImage;
}
//Getters
//--------------------------------------------------------------------------
/**
* Gets the comment associated with the data set.
* This comment will be written to the CSV log file when saving the statistics.
*
* This is appropriate for discussing the distribution of the data, dimensions of the data, etc.
* @return message about the data set
*/
public String getDatasetMessage(){
return datasetMessage;
}
/**
* Gets the general comment associated with the profile.
* This comment will be written to the CSV log file when saving the statistics.
*
* This is appropriate for discussing the parameters of the renderer, etc.
* @return general message about the profiling results
*/
public String getSaveMessage(){
return saveMessage;
}
/**
* Gets the author associated with the profile.
* This comment will be written to the CSV log file when saving the statistics.
* <p>
* This is appropriate for stating the user who profiled.
* This can help provide understanding in different results due
* to computer hardware differences.
*
* @return author for the profiling
*/
public String getAuthorMessage(){
return this.authorMessage;
}
/**
* Gets the image to be saved.
* The image is one of the images rendered during profiling.
* This field is not written to the comments of the CSV log file.
* @return image of the graph to be saved
*/
public BufferedImage getSaveImage(){
return saveImage;
}
//--------------------------------------------------------------------------
//FORMATS FOR OUTPUT FILE
//--------------------------------------------------------------------------
/**
* Gets the .CSV formatted header for the:
* (title row)
* <ol>
* <li>Dataset comments</li>
* <li>Author</li>
* <li>General Message</li>
* </ol>
*
* @return the header for the general comments output
*/
private String[] getSaveOutputHeader(){
return new String[]{
"Dataset Comments",
"Author",
"General Message"
};
}
/**
* Gets the .CSV formatted record for the:
* (information row)
* <ol>
* <li>Dataset comments</li>
* <li>Author</li>
* <li>General Message</li>
* </ol>
*
* @return the record for the general comments output
*/
private String[] getSaveOutputMessage(){
String quote = "\"";
String delim = ",";
return new String[]{
getDatasetMessage(),
getAuthorMessage(),
getSaveMessage()
};
}
/**
* Gets the .CSV formatted header for the:
* (title row)
* <ol>
* <li>Java Virtual Machine Version</li>
* <li>Available Memory</li>
* <li>Total RAM</li>
* <li>Operating System Name</li>
* <li>Operating System Version</li>
* </ol>
*
* @return the header for the hardware comments output
*/
private String[] getHardwareTitle(){
return new String[]{
"JVM Version",
"Available Memory (GB)",
"RAM (GB)",
"OS",
"OS Version"
};
}
/**
* Gets the .CSV formatted record for the:
* (information row)
* <ol>
* <li>Java Virtual Machine Version</li>
* <li>Available Memory</li>
* <li>Total RAM</li>
* <li>Operating System Name</li>
* <li>Operating System Version</li>
* </ol>
*
* @return the record for the hardware comments output
*/
private String[] getHardwareMessage(){
//Get Environment Properties
String javaVersion = System.getProperty("java.version");
String os = System.getProperty("os.name");
String osVersion = System.getProperty("os.version");
//Format
javaVersion = javaVersion == null ? "": javaVersion;
os = os == null ? "": os;
osVersion = osVersion == null ? "": osVersion;
//Memory
double runtime = Runtime.getRuntime().maxMemory() / SaveSettings.BYTES_TO_GB;
double memorySize = ((com.sun.management.OperatingSystemMXBean) ManagementFactory.getOperatingSystemMXBean()).getTotalPhysicalMemorySize() / SaveSettings.BYTES_TO_GB;
return new String[]{
javaVersion,
String.format("%.3f", runtime),
String.format("%.3f", memorySize),
os,
osVersion
};
}
//--------------------------------------------------------------------------
//COMBINED FORMATS FOR OUTPUT FILE
//--------------------------------------------------------------------------
/**
* List of headers for the data members.
* @return header data fields
*/
@Override
public Object[] getTitle() {
Object[] s = getSaveOutputHeader();
Object[] h = getHardwareTitle();
Object[] entries = new Object[s.length + h.length];
System.arraycopy(s, 0, entries, 0, s.length);
System.arraycopy(h, 0, entries, s.length, h.length);
return entries;
}
/**
* List of headers for the data members.
* @return header data fields
*/
@Override
public Object[] getOutput() {
Object[] s = getSaveOutputMessage();
Object[] h = getHardwareMessage();
Object[] entries = new Object[s.length + h.length];
System.arraycopy(s, 0, entries, 0, s.length);
System.arraycopy(h, 0, entries, s.length, h.length);
return entries;
}
//--------------------------------------------------------------------------
}
| {
"content_hash": "565e38baa5d0895b3813532637901676",
"timestamp": "",
"source": "github",
"line_count": 288,
"max_line_length": 174,
"avg_line_length": 29.555555555555557,
"alnum_prop": 0.5465225563909775,
"repo_name": "ControlSystemStudio/diirt",
"id": "80030f3e6e75ddae8b5cbf6cd93be0117a07474e",
"size": "8653",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "graphene/graphene-profile/src/main/java/org/diirt/graphene/profile/settings/SaveSettings.java",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "Batchfile",
"bytes": "314"
},
{
"name": "CSS",
"bytes": "75261"
},
{
"name": "GAP",
"bytes": "5847"
},
{
"name": "HTML",
"bytes": "417393"
},
{
"name": "Java",
"bytes": "4798256"
},
{
"name": "JavaScript",
"bytes": "1745584"
},
{
"name": "Shell",
"bytes": "3095"
}
],
"symlink_target": ""
} |
package org.elasticsearch.cluster.routing.allocation.allocator;
import org.apache.lucene.util.ArrayUtil;
import org.apache.lucene.util.IntroSorter;
import org.elasticsearch.cluster.metadata.IndexMetaData;
import org.elasticsearch.cluster.metadata.MetaData;
import org.elasticsearch.cluster.node.DiscoveryNode;
import org.elasticsearch.cluster.routing.RoutingNode;
import org.elasticsearch.cluster.routing.RoutingNodes;
import org.elasticsearch.cluster.routing.ShardRouting;
import org.elasticsearch.cluster.routing.ShardRoutingState;
import org.elasticsearch.cluster.routing.allocation.RoutingAllocation;
import org.elasticsearch.cluster.routing.allocation.decider.AllocationDeciders;
import org.elasticsearch.cluster.routing.allocation.decider.Decision;
import org.elasticsearch.cluster.routing.allocation.decider.Decision.Type;
import org.elasticsearch.common.collect.Tuple;
import org.elasticsearch.common.component.AbstractComponent;
import org.elasticsearch.common.inject.Inject;
import org.elasticsearch.common.logging.ESLogger;
import org.elasticsearch.common.settings.ClusterSettings;
import org.elasticsearch.common.settings.Setting;
import org.elasticsearch.common.settings.Setting.Property;
import org.elasticsearch.common.settings.Settings;
import org.elasticsearch.gateway.PriorityComparator;
import java.util.Collections;
import java.util.Comparator;
import java.util.HashMap;
import java.util.HashSet;
import java.util.IdentityHashMap;
import java.util.Iterator;
import java.util.Map;
import java.util.Set;
import static org.elasticsearch.cluster.routing.ShardRoutingState.RELOCATING;
/**
* The {@link BalancedShardsAllocator} re-balances the nodes allocations
* within an cluster based on a {@link WeightFunction}. The clusters balance is defined by four parameters which can be set
* in the cluster update API that allows changes in real-time:
* <ul><li><code>cluster.routing.allocation.balance.shard</code> - The <b>shard balance</b> defines the weight factor
* for shards allocated on a {@link RoutingNode}</li>
* <li><code>cluster.routing.allocation.balance.index</code> - The <b>index balance</b> defines a factor to the number
* of {@link org.elasticsearch.cluster.routing.ShardRouting}s per index allocated on a specific node</li>
* <li><code>cluster.routing.allocation.balance.threshold</code> - A <b>threshold</b> to set the minimal optimization
* value of operations that should be performed</li>
* </ul>
* <p>
* These parameters are combined in a {@link WeightFunction} that allows calculation of node weights which
* are used to re-balance shards based on global as well as per-index factors.
*/
public class BalancedShardsAllocator extends AbstractComponent implements ShardsAllocator {
public static final Setting<Float> INDEX_BALANCE_FACTOR_SETTING =
Setting.floatSetting("cluster.routing.allocation.balance.index", 0.55f, Property.Dynamic, Property.NodeScope);
public static final Setting<Float> SHARD_BALANCE_FACTOR_SETTING =
Setting.floatSetting("cluster.routing.allocation.balance.shard", 0.45f, Property.Dynamic, Property.NodeScope);
public static final Setting<Float> THRESHOLD_SETTING =
Setting.floatSetting("cluster.routing.allocation.balance.threshold", 1.0f, 0.0f,
Property.Dynamic, Property.NodeScope);
private volatile WeightFunction weightFunction;
private volatile float threshold;
public BalancedShardsAllocator(Settings settings) {
this(settings, new ClusterSettings(settings, ClusterSettings.BUILT_IN_CLUSTER_SETTINGS));
}
@Inject
public BalancedShardsAllocator(Settings settings, ClusterSettings clusterSettings) {
super(settings);
setWeightFunction(INDEX_BALANCE_FACTOR_SETTING.get(settings), SHARD_BALANCE_FACTOR_SETTING.get(settings));
setThreshold(THRESHOLD_SETTING.get(settings));
clusterSettings.addSettingsUpdateConsumer(INDEX_BALANCE_FACTOR_SETTING, SHARD_BALANCE_FACTOR_SETTING, this::setWeightFunction);
clusterSettings.addSettingsUpdateConsumer(THRESHOLD_SETTING, this::setThreshold);
}
private void setWeightFunction(float indexBalance, float shardBalanceFactor) {
weightFunction = new WeightFunction(indexBalance, shardBalanceFactor);
}
private void setThreshold(float threshold) {
this.threshold = threshold;
}
@Override
public Map<DiscoveryNode, Float> weighShard(RoutingAllocation allocation, ShardRouting shard) {
final Balancer balancer = new Balancer(logger, allocation, weightFunction, threshold);
return balancer.weighShard(shard);
}
@Override
public boolean allocate(RoutingAllocation allocation) {
if (allocation.routingNodes().size() == 0) {
/* with no nodes this is pointless */
return false;
}
final Balancer balancer = new Balancer(logger, allocation, weightFunction, threshold);
boolean changed = balancer.allocateUnassigned();
changed |= balancer.moveShards();
changed |= balancer.balance();
return changed;
}
/**
* Returns the currently configured delta threshold
*/
public float getThreshold() {
return threshold;
}
/**
* Returns the index related weight factor.
*/
public float getIndexBalance() {
return weightFunction.indexBalance;
}
/**
* Returns the shard related weight factor.
*/
public float getShardBalance() {
return weightFunction.shardBalance;
}
/**
* This class is the primary weight function used to create balanced over nodes and shards in the cluster.
* Currently this function has 3 properties:
* <ul>
* <li><code>index balance</code> - balance property over shards per index</li>
* <li><code>shard balance</code> - balance property over shards per cluster</li>
* </ul>
* <p>
* Each of these properties are expressed as factor such that the properties factor defines the relative importance of the property for the
* weight function. For example if the weight function should calculate the weights only based on a global (shard) balance the index balance
* can be set to <tt>0.0</tt> and will in turn have no effect on the distribution.
* </p>
* The weight per index is calculated based on the following formula:
* <ul>
* <li>
* <code>weight<sub>index</sub>(node, index) = indexBalance * (node.numShards(index) - avgShardsPerNode(index))</code>
* </li>
* <li>
* <code>weight<sub>node</sub>(node, index) = shardBalance * (node.numShards() - avgShardsPerNode)</code>
* </li>
* </ul>
* <code>weight(node, index) = weight<sub>index</sub>(node, index) + weight<sub>node</sub>(node, index)</code>
*/
public static class WeightFunction {
private final float indexBalance;
private final float shardBalance;
private final float theta0;
private final float theta1;
public WeightFunction(float indexBalance, float shardBalance) {
float sum = indexBalance + shardBalance;
if (sum <= 0.0f) {
throw new IllegalArgumentException("Balance factors must sum to a value > 0 but was: " + sum);
}
theta0 = shardBalance / sum;
theta1 = indexBalance / sum;
this.indexBalance = indexBalance;
this.shardBalance = shardBalance;
}
public float weight(Balancer balancer, ModelNode node, String index) {
return weight(balancer, node, index, 0);
}
public float weightShardAdded(Balancer balancer, ModelNode node, String index) {
return weight(balancer, node, index, 1);
}
public float weightShardRemoved(Balancer balancer, ModelNode node, String index) {
return weight(balancer, node, index, -1);
}
private float weight(Balancer balancer, ModelNode node, String index, int numAdditionalShards) {
final float weightShard = node.numShards() + numAdditionalShards - balancer.avgShardsPerNode();
final float weightIndex = node.numShards(index) + numAdditionalShards - balancer.avgShardsPerNode(index);
return theta0 * weightShard + theta1 * weightIndex;
}
}
/**
* A {@link Balancer}
*/
public static class Balancer {
private final ESLogger logger;
private final Map<String, ModelNode> nodes = new HashMap<>();
private final RoutingAllocation allocation;
private final RoutingNodes routingNodes;
private final WeightFunction weight;
private final float threshold;
private final MetaData metaData;
private final float avgShardsPerNode;
public Balancer(ESLogger logger, RoutingAllocation allocation, WeightFunction weight, float threshold) {
this.logger = logger;
this.allocation = allocation;
this.weight = weight;
this.threshold = threshold;
this.routingNodes = allocation.routingNodes();
this.metaData = allocation.metaData();
avgShardsPerNode = ((float) metaData.getTotalNumberOfShards()) / routingNodes.size();
buildModelFromAssigned();
}
/**
* Returns an array view on the nodes in the balancer. Nodes should not be removed from this list.
*/
private ModelNode[] nodesArray() {
return nodes.values().toArray(new ModelNode[nodes.size()]);
}
/**
* Returns the average of shards per node for the given index
*/
public float avgShardsPerNode(String index) {
return ((float) metaData.index(index).getTotalNumberOfShards()) / nodes.size();
}
/**
* Returns the global average of shards per node
*/
public float avgShardsPerNode() {
return avgShardsPerNode;
}
/**
* Returns a new {@link NodeSorter} that sorts the nodes based on their
* current weight with respect to the index passed to the sorter. The
* returned sorter is not sorted. Use {@link NodeSorter#reset(String)}
* to sort based on an index.
*/
private NodeSorter newNodeSorter() {
return new NodeSorter(nodesArray(), weight, this);
}
private static float absDelta(float lower, float higher) {
assert higher >= lower : higher + " lt " + lower +" but was expected to be gte";
return Math.abs(higher - lower);
}
private static boolean lessThan(float delta, float threshold) {
/* deltas close to the threshold are "rounded" to the threshold manually
to prevent floating point problems if the delta is very close to the
threshold ie. 1.000000002 which can trigger unnecessary balance actions*/
return delta <= (threshold + 0.001f);
}
/**
* Balances the nodes on the cluster model according to the weight function.
* The actual balancing is delegated to {@link #balanceByWeights()}
*
* @return <code>true</code> if the current configuration has been
* changed, otherwise <code>false</code>
*/
private boolean balance() {
if (logger.isTraceEnabled()) {
logger.trace("Start balancing cluster");
}
if (allocation.hasPendingAsyncFetch()) {
/*
* see https://github.com/elastic/elasticsearch/issues/14387
* if we allow rebalance operations while we are still fetching shard store data
* we might end up with unnecessary rebalance operations which can be super confusion/frustrating
* since once the fetches come back we might just move all the shards back again.
* Therefore we only do a rebalance if we have fetched all information.
*/
logger.debug("skipping rebalance due to in-flight shard/store fetches");
return false;
}
if (allocation.deciders().canRebalance(allocation).type() != Type.YES) {
logger.trace("skipping rebalance as it is disabled");
return false;
}
if (nodes.size() < 2) { /* skip if we only have one node */
logger.trace("skipping rebalance as single node only");
return false;
}
return balanceByWeights();
}
public Map<DiscoveryNode, Float> weighShard(ShardRouting shard) {
final NodeSorter sorter = newNodeSorter();
final ModelNode[] modelNodes = sorter.modelNodes;
final float[] weights = sorter.weights;
buildWeightOrderedIndices(sorter);
Map<DiscoveryNode, Float> nodes = new HashMap<>(modelNodes.length);
float currentNodeWeight = 0.0f;
for (int i = 0; i < modelNodes.length; i++) {
if (modelNodes[i].getNodeId().equals(shard.currentNodeId())) {
// If a node was found with the shard, use that weight instead of 0.0
currentNodeWeight = weights[i];
break;
}
}
for (int i = 0; i < modelNodes.length; i++) {
final float delta = currentNodeWeight - weights[i];
nodes.put(modelNodes[i].getRoutingNode().node(), delta);
}
return nodes;
}
/**
* Balances the nodes on the cluster model according to the weight
* function. The configured threshold is the minimum delta between the
* weight of the maximum node and the minimum node according to the
* {@link WeightFunction}. This weight is calculated per index to
* distribute shards evenly per index. The balancer tries to relocate
* shards only if the delta exceeds the threshold. If the default case
* the threshold is set to <tt>1.0</tt> to enforce gaining relocation
* only, or in other words relocations that move the weight delta closer
* to <tt>0.0</tt>
*
* @return <code>true</code> if the current configuration has been
* changed, otherwise <code>false</code>
*/
private boolean balanceByWeights() {
boolean changed = false;
final NodeSorter sorter = newNodeSorter();
final AllocationDeciders deciders = allocation.deciders();
final ModelNode[] modelNodes = sorter.modelNodes;
final float[] weights = sorter.weights;
for (String index : buildWeightOrderedIndices(sorter)) {
IndexMetaData indexMetaData = metaData.index(index);
// find nodes that have a shard of this index or where shards of this index are allowed to stay
// move these nodes to the front of modelNodes so that we can only balance based on these nodes
int relevantNodes = 0;
for (int i = 0; i < modelNodes.length; i++) {
ModelNode modelNode = modelNodes[i];
if (modelNode.getIndex(index) != null
|| deciders.canAllocate(indexMetaData, modelNode.getRoutingNode(), allocation).type() != Type.NO) {
// swap nodes at position i and relevantNodes
modelNodes[i] = modelNodes[relevantNodes];
modelNodes[relevantNodes] = modelNode;
relevantNodes++;
}
}
if (relevantNodes < 2) {
continue;
}
sorter.reset(index, 0, relevantNodes);
int lowIdx = 0;
int highIdx = relevantNodes - 1;
while (true) {
final ModelNode minNode = modelNodes[lowIdx];
final ModelNode maxNode = modelNodes[highIdx];
advance_range:
if (maxNode.numShards(index) > 0) {
final float delta = absDelta(weights[lowIdx], weights[highIdx]);
if (lessThan(delta, threshold)) {
if (lowIdx > 0 && highIdx-1 > 0 // is there a chance for a higher delta?
&& (absDelta(weights[0], weights[highIdx-1]) > threshold) // check if we need to break at all
) {
/* This is a special case if allocations from the "heaviest" to the "lighter" nodes is not possible
* due to some allocation decider restrictions like zone awareness. if one zone has for instance
* less nodes than another zone. so one zone is horribly overloaded from a balanced perspective but we
* can't move to the "lighter" shards since otherwise the zone would go over capacity.
*
* This break jumps straight to the condition below were we start moving from the high index towards
* the low index to shrink the window we are considering for balance from the other direction.
* (check shrinking the window from MAX to MIN)
* See #3580
*/
break advance_range;
}
if (logger.isTraceEnabled()) {
logger.trace("Stop balancing index [{}] min_node [{}] weight: [{}] max_node [{}] weight: [{}] delta: [{}]",
index, maxNode.getNodeId(), weights[highIdx], minNode.getNodeId(), weights[lowIdx], delta);
}
break;
}
if (logger.isTraceEnabled()) {
logger.trace("Balancing from node [{}] weight: [{}] to node [{}] weight: [{}] delta: [{}]",
maxNode.getNodeId(), weights[highIdx], minNode.getNodeId(), weights[lowIdx], delta);
}
/* pass the delta to the replication function to prevent relocations that only swap the weights of the two nodes.
* a relocation must bring us closer to the balance if we only achieve the same delta the relocation is useless */
if (tryRelocateShard(minNode, maxNode, index, delta)) {
/*
* TODO we could be a bit smarter here, we don't need to fully sort necessarily
* we could just find the place to insert linearly but the win might be minor
* compared to the added complexity
*/
weights[lowIdx] = sorter.weight(modelNodes[lowIdx]);
weights[highIdx] = sorter.weight(modelNodes[highIdx]);
sorter.sort(0, relevantNodes);
lowIdx = 0;
highIdx = relevantNodes - 1;
changed = true;
continue;
}
}
if (lowIdx < highIdx - 1) {
/* Shrinking the window from MIN to MAX
* we can't move from any shard from the min node lets move on to the next node
* and see if the threshold still holds. We either don't have any shard of this
* index on this node of allocation deciders prevent any relocation.*/
lowIdx++;
} else if (lowIdx > 0) {
/* Shrinking the window from MAX to MIN
* now we go max to min since obviously we can't move anything to the max node
* lets pick the next highest */
lowIdx = 0;
highIdx--;
} else {
/* we are done here, we either can't relocate anymore or we are balanced */
break;
}
}
}
return changed;
}
/**
* This builds a initial index ordering where the indices are returned
* in most unbalanced first. We need this in order to prevent over
* allocations on added nodes from one index when the weight parameters
* for global balance overrule the index balance at an intermediate
* state. For example this can happen if we have 3 nodes and 3 indices
* with 3 shards and 1 shard. At the first stage all three nodes hold
* 2 shard for each index. now we add another node and the first index
* is balanced moving 3 two of the nodes over to the new node since it
* has no shards yet and global balance for the node is way below
* average. To re-balance we need to move shards back eventually likely
* to the nodes we relocated them from.
*/
private String[] buildWeightOrderedIndices(NodeSorter sorter) {
final String[] indices = allocation.routingTable().indicesRouting().keys().toArray(String.class);
final float[] deltas = new float[indices.length];
for (int i = 0; i < deltas.length; i++) {
sorter.reset(indices[i]);
deltas[i] = sorter.delta();
}
new IntroSorter() {
float pivotWeight;
@Override
protected void swap(int i, int j) {
final String tmpIdx = indices[i];
indices[i] = indices[j];
indices[j] = tmpIdx;
final float tmpDelta = deltas[i];
deltas[i] = deltas[j];
deltas[j] = tmpDelta;
}
@Override
protected int compare(int i, int j) {
return Float.compare(deltas[j], deltas[i]);
}
@Override
protected void setPivot(int i) {
pivotWeight = deltas[i];
}
@Override
protected int comparePivot(int j) {
return Float.compare(deltas[j], pivotWeight);
}
}.sort(0, deltas.length);
return indices;
}
/**
* Move started shards that can not be allocated to a node anymore
*
* For each shard to be moved this function executes a move operation
* to the minimal eligible node with respect to the
* weight function. If a shard is moved the shard will be set to
* {@link ShardRoutingState#RELOCATING} and a shadow instance of this
* shard is created with an incremented version in the state
* {@link ShardRoutingState#INITIALIZING}.
*
* @return <code>true</code> if the allocation has changed, otherwise <code>false</code>
*/
public boolean moveShards() {
// Iterate over the started shards interleaving between nodes, and check if they can remain. In the presence of throttling
// shard movements, the goal of this iteration order is to achieve a fairer movement of shards from the nodes that are
// offloading the shards.
boolean changed = false;
final NodeSorter sorter = newNodeSorter();
for (Iterator<ShardRouting> it = allocation.routingNodes().nodeInterleavedShardIterator(); it.hasNext(); ) {
ShardRouting shardRouting = it.next();
// we can only move started shards...
if (shardRouting.started()) {
final ModelNode sourceNode = nodes.get(shardRouting.currentNodeId());
assert sourceNode != null && sourceNode.containsShard(shardRouting);
RoutingNode routingNode = sourceNode.getRoutingNode();
Decision decision = allocation.deciders().canRemain(shardRouting, routingNode, allocation);
if (decision.type() == Decision.Type.NO) {
changed |= moveShard(sorter, shardRouting, sourceNode, routingNode);
}
}
}
return changed;
}
/**
* Move started shard to the minimal eligible node with respect to the weight function
*
* @return <code>true</code> if the shard was moved successfully, otherwise <code>false</code>
*/
private boolean moveShard(NodeSorter sorter, ShardRouting shardRouting, ModelNode sourceNode, RoutingNode routingNode) {
logger.debug("[{}][{}] allocated on [{}], but can no longer be allocated on it, moving...", shardRouting.index(), shardRouting.id(), routingNode.node());
sorter.reset(shardRouting.getIndexName());
/*
* the sorter holds the minimum weight node first for the shards index.
* We now walk through the nodes until we find a node to allocate the shard.
* This is not guaranteed to be balanced after this operation we still try best effort to
* allocate on the minimal eligible node.
*/
for (ModelNode currentNode : sorter.modelNodes) {
if (currentNode != sourceNode) {
RoutingNode target = currentNode.getRoutingNode();
// don't use canRebalance as we want hard filtering rules to apply. See #17698
Decision allocationDecision = allocation.deciders().canAllocate(shardRouting, target, allocation);
if (allocationDecision.type() == Type.YES) { // TODO maybe we can respect throttling here too?
sourceNode.removeShard(shardRouting);
Tuple<ShardRouting, ShardRouting> relocatingShards = routingNodes.relocate(shardRouting, target.nodeId(), allocation.clusterInfo().getShardSize(shardRouting, ShardRouting.UNAVAILABLE_EXPECTED_SHARD_SIZE));
currentNode.addShard(relocatingShards.v2());
if (logger.isTraceEnabled()) {
logger.trace("Moved shard [{}] to node [{}]", shardRouting, routingNode.node());
}
return true;
}
}
}
logger.debug("[{}][{}] can't move", shardRouting.index(), shardRouting.id());
return false;
}
/**
* Builds the internal model from all shards in the given
* {@link Iterable}. All shards in the {@link Iterable} must be assigned
* to a node. This method will skip shards in the state
* {@link ShardRoutingState#RELOCATING} since each relocating shard has
* a shadow shard in the state {@link ShardRoutingState#INITIALIZING}
* on the target node which we respect during the allocation / balancing
* process. In short, this method recreates the status-quo in the cluster.
*/
private void buildModelFromAssigned() {
for (RoutingNode rn : routingNodes) {
ModelNode node = new ModelNode(rn);
nodes.put(rn.nodeId(), node);
for (ShardRouting shard : rn) {
assert rn.nodeId().equals(shard.currentNodeId());
/* we skip relocating shards here since we expect an initializing shard with the same id coming in */
if (shard.state() != RELOCATING) {
node.addShard(shard);
if (logger.isTraceEnabled()) {
logger.trace("Assigned shard [{}] to node [{}]", shard, node.getNodeId());
}
}
}
}
}
/**
* Allocates all given shards on the minimal eligible node for the shards index
* with respect to the weight function. All given shards must be unassigned.
* @return <code>true</code> if the current configuration has been
* changed, otherwise <code>false</code>
*/
private boolean allocateUnassigned() {
RoutingNodes.UnassignedShards unassigned = routingNodes.unassigned();
assert !nodes.isEmpty();
if (logger.isTraceEnabled()) {
logger.trace("Start allocating unassigned shards");
}
if (unassigned.isEmpty()) {
return false;
}
boolean changed = false;
/*
* TODO: We could be smarter here and group the shards by index and then
* use the sorter to save some iterations.
*/
final AllocationDeciders deciders = allocation.deciders();
final PriorityComparator secondaryComparator = PriorityComparator.getAllocationComparator(allocation);
final Comparator<ShardRouting> comparator = (o1, o2) -> {
if (o1.primary() ^ o2.primary()) {
return o1.primary() ? -1 : o2.primary() ? 1 : 0;
}
final int indexCmp;
if ((indexCmp = o1.getIndexName().compareTo(o2.getIndexName())) == 0) {
return o1.getId() - o2.getId();
}
// this comparator is more expensive than all the others up there
// that's why it's added last even though it could be easier to read
// if we'd apply it earlier. this comparator will only differentiate across
// indices all shards of the same index is treated equally.
final int secondary = secondaryComparator.compare(o1, o2);
return secondary == 0 ? indexCmp : secondary;
};
/*
* we use 2 arrays and move replicas to the second array once we allocated an identical
* replica in the current iteration to make sure all indices get allocated in the same manner.
* The arrays are sorted by primaries first and then by index and shard ID so a 2 indices with 2 replica and 1 shard would look like:
* [(0,P,IDX1), (0,P,IDX2), (0,R,IDX1), (0,R,IDX1), (0,R,IDX2), (0,R,IDX2)]
* if we allocate for instance (0, R, IDX1) we move the second replica to the secondary array and proceed with
* the next replica. If we could not find a node to allocate (0,R,IDX1) we move all it's replicas to ignoreUnassigned.
*/
ShardRouting[] primary = unassigned.drain();
ShardRouting[] secondary = new ShardRouting[primary.length];
int secondaryLength = 0;
int primaryLength = primary.length;
ArrayUtil.timSort(primary, comparator);
final Set<ModelNode> throttledNodes = Collections.newSetFromMap(new IdentityHashMap<>());
do {
for (int i = 0; i < primaryLength; i++) {
ShardRouting shard = primary[i];
if (!shard.primary()) {
boolean drop = deciders.canAllocate(shard, allocation).type() == Type.NO;
if (drop) {
unassigned.ignoreShard(shard);
while(i < primaryLength-1 && comparator.compare(primary[i], primary[i+1]) == 0) {
unassigned.ignoreShard(primary[++i]);
}
continue;
} else {
while(i < primaryLength-1 && comparator.compare(primary[i], primary[i+1]) == 0) {
secondary[secondaryLength++] = primary[++i];
}
}
}
assert !shard.assignedToNode() : shard;
/* find an node with minimal weight we can allocate on*/
float minWeight = Float.POSITIVE_INFINITY;
ModelNode minNode = null;
Decision decision = null;
if (throttledNodes.size() < nodes.size()) {
/* Don't iterate over an identity hashset here the
* iteration order is different for each run and makes testing hard */
for (ModelNode node : nodes.values()) {
if (throttledNodes.contains(node)) {
continue;
}
if (!node.containsShard(shard)) {
// simulate weight if we would add shard to node
float currentWeight = weight.weightShardAdded(this, node, shard.getIndexName());
/*
* Unless the operation is not providing any gains we
* don't check deciders
*/
if (currentWeight <= minWeight) {
Decision currentDecision = deciders.canAllocate(shard, node.getRoutingNode(), allocation);
NOUPDATE:
if (currentDecision.type() == Type.YES || currentDecision.type() == Type.THROTTLE) {
if (currentWeight == minWeight) {
/* we have an equal weight tie breaking:
* 1. if one decision is YES prefer it
* 2. prefer the node that holds the primary for this index with the next id in the ring ie.
* for the 3 shards 2 replica case we try to build up:
* 1 2 0
* 2 0 1
* 0 1 2
* such that if we need to tie-break we try to prefer the node holding a shard with the minimal id greater
* than the id of the shard we need to assign. This works find when new indices are created since
* primaries are added first and we only add one shard set a time in this algorithm.
*/
if (currentDecision.type() == decision.type()) {
final int repId = shard.id();
final int nodeHigh = node.highestPrimary(shard.index().getName());
final int minNodeHigh = minNode.highestPrimary(shard.getIndexName());
if ((((nodeHigh > repId && minNodeHigh > repId) || (nodeHigh < repId && minNodeHigh < repId)) && (nodeHigh < minNodeHigh))
|| (nodeHigh > minNodeHigh && nodeHigh > repId && minNodeHigh < repId)) {
minNode = node;
minWeight = currentWeight;
decision = currentDecision;
} else {
break NOUPDATE;
}
} else if (currentDecision.type() != Type.YES) {
break NOUPDATE;
}
}
minNode = node;
minWeight = currentWeight;
decision = currentDecision;
}
}
}
}
}
assert decision != null && minNode != null || decision == null && minNode == null;
if (minNode != null) {
long shardSize = allocation.clusterInfo().getShardSize(shard, ShardRouting.UNAVAILABLE_EXPECTED_SHARD_SIZE);
if (decision.type() == Type.YES) {
if (logger.isTraceEnabled()) {
logger.trace("Assigned shard [{}] to [{}]", shard, minNode.getNodeId());
}
shard = routingNodes.initialize(shard, minNode.getNodeId(), null, shardSize);
minNode.addShard(shard);
changed = true;
continue; // don't add to ignoreUnassigned
} else {
minNode.addShard(shard.initialize(minNode.getNodeId(), null, shardSize));
final RoutingNode node = minNode.getRoutingNode();
if (deciders.canAllocate(node, allocation).type() != Type.YES) {
if (logger.isTraceEnabled()) {
logger.trace("Can not allocate on node [{}] remove from round decision [{}]", node, decision.type());
}
throttledNodes.add(minNode);
}
}
if (logger.isTraceEnabled()) {
logger.trace("No eligible node found to assign shard [{}] decision [{}]", shard, decision.type());
}
} else if (logger.isTraceEnabled()) {
logger.trace("No Node found to assign shard [{}]", shard);
}
unassigned.ignoreShard(shard);
if (!shard.primary()) { // we could not allocate it and we are a replica - check if we can ignore the other replicas
while(secondaryLength > 0 && comparator.compare(shard, secondary[secondaryLength-1]) == 0) {
unassigned.ignoreShard(secondary[--secondaryLength]);
}
}
}
primaryLength = secondaryLength;
ShardRouting[] tmp = primary;
primary = secondary;
secondary = tmp;
secondaryLength = 0;
} while (primaryLength > 0);
// clear everything we have either added it or moved to ignoreUnassigned
return changed;
}
/**
* Tries to find a relocation from the max node to the minimal node for an arbitrary shard of the given index on the
* balance model. Iff this method returns a <code>true</code> the relocation has already been executed on the
* simulation model as well as on the cluster.
*/
private boolean tryRelocateShard(ModelNode minNode, ModelNode maxNode, String idx, float minCost) {
final ModelIndex index = maxNode.getIndex(idx);
Decision decision = null;
if (index != null) {
if (logger.isTraceEnabled()) {
logger.trace("Try relocating shard for index index [{}] from node [{}] to node [{}]", idx, maxNode.getNodeId(),
minNode.getNodeId());
}
ShardRouting candidate = null;
final AllocationDeciders deciders = allocation.deciders();
for (ShardRouting shard : index) {
if (shard.started()) {
// skip initializing, unassigned and relocating shards we can't relocate them anyway
Decision allocationDecision = deciders.canAllocate(shard, minNode.getRoutingNode(), allocation);
Decision rebalanceDecision = deciders.canRebalance(shard, allocation);
if (((allocationDecision.type() == Type.YES) || (allocationDecision.type() == Type.THROTTLE))
&& ((rebalanceDecision.type() == Type.YES) || (rebalanceDecision.type() == Type.THROTTLE))) {
if (maxNode.containsShard(shard)) {
// simulate moving shard from maxNode to minNode
final float delta = weight.weightShardAdded(this, minNode, idx) - weight.weightShardRemoved(this, maxNode, idx);
if (delta < minCost ||
(candidate != null && delta == minCost && candidate.id() > shard.id())) {
/* this last line is a tie-breaker to make the shard allocation alg deterministic
* otherwise we rely on the iteration order of the index.getAllShards() which is a set.*/
minCost = delta;
candidate = shard;
decision = new Decision.Multi().add(allocationDecision).add(rebalanceDecision);
}
}
}
}
}
if (candidate != null) {
/* allocate on the model even if not throttled */
maxNode.removeShard(candidate);
long shardSize = allocation.clusterInfo().getShardSize(candidate, ShardRouting.UNAVAILABLE_EXPECTED_SHARD_SIZE);
if (decision.type() == Type.YES) { /* only allocate on the cluster if we are not throttled */
if (logger.isTraceEnabled()) {
logger.trace("Relocate shard [{}] from node [{}] to node [{}]", candidate, maxNode.getNodeId(),
minNode.getNodeId());
}
/* now allocate on the cluster */
minNode.addShard(routingNodes.relocate(candidate, minNode.getNodeId(), shardSize).v1());
return true;
} else {
assert decision.type() == Type.THROTTLE;
minNode.addShard(candidate.relocate(minNode.getNodeId(), shardSize));
}
}
}
if (logger.isTraceEnabled()) {
logger.trace("Couldn't find shard to relocate from node [{}] to node [{}] allocation decision [{}]", maxNode.getNodeId(),
minNode.getNodeId(), decision == null ? "NO" : decision.type().name());
}
return false;
}
}
static class ModelNode implements Iterable<ModelIndex> {
private final Map<String, ModelIndex> indices = new HashMap<>();
private int numShards = 0;
private final RoutingNode routingNode;
public ModelNode(RoutingNode routingNode) {
this.routingNode = routingNode;
}
public ModelIndex getIndex(String indexId) {
return indices.get(indexId);
}
public String getNodeId() {
return routingNode.nodeId();
}
public RoutingNode getRoutingNode() {
return routingNode;
}
public int numShards() {
return numShards;
}
public int numShards(String idx) {
ModelIndex index = indices.get(idx);
return index == null ? 0 : index.numShards();
}
public int highestPrimary(String index) {
ModelIndex idx = indices.get(index);
if (idx != null) {
return idx.highestPrimary();
}
return -1;
}
public void addShard(ShardRouting shard) {
ModelIndex index = indices.get(shard.getIndexName());
if (index == null) {
index = new ModelIndex(shard.getIndexName());
indices.put(index.getIndexId(), index);
}
index.addShard(shard);
numShards++;
}
public void removeShard(ShardRouting shard) {
ModelIndex index = indices.get(shard.getIndexName());
if (index != null) {
index.removeShard(shard);
if (index.numShards() == 0) {
indices.remove(shard.getIndexName());
}
}
numShards--;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("Node(").append(routingNode.nodeId()).append(")");
return sb.toString();
}
@Override
public Iterator<ModelIndex> iterator() {
return indices.values().iterator();
}
public boolean containsShard(ShardRouting shard) {
ModelIndex index = getIndex(shard.getIndexName());
return index == null ? false : index.containsShard(shard);
}
}
static final class ModelIndex implements Iterable<ShardRouting> {
private final String id;
private final Set<ShardRouting> shards = new HashSet<>(4); // expect few shards of same index to be allocated on same node
private int highestPrimary = -1;
public ModelIndex(String id) {
this.id = id;
}
public int highestPrimary() {
if (highestPrimary == -1) {
int maxId = -1;
for (ShardRouting shard : shards) {
if (shard.primary()) {
maxId = Math.max(maxId, shard.id());
}
}
return highestPrimary = maxId;
}
return highestPrimary;
}
public String getIndexId() {
return id;
}
public int numShards() {
return shards.size();
}
@Override
public Iterator<ShardRouting> iterator() {
return shards.iterator();
}
public void removeShard(ShardRouting shard) {
highestPrimary = -1;
assert shards.contains(shard) : "Shard not allocated on current node: " + shard;
shards.remove(shard);
}
public void addShard(ShardRouting shard) {
highestPrimary = -1;
assert !shards.contains(shard) : "Shard already allocated on current node: " + shard;
shards.add(shard);
}
public boolean containsShard(ShardRouting shard) {
return shards.contains(shard);
}
}
static final class NodeSorter extends IntroSorter {
final ModelNode[] modelNodes;
/* the nodes weights with respect to the current weight function / index */
final float[] weights;
private final WeightFunction function;
private String index;
private final Balancer balancer;
private float pivotWeight;
public NodeSorter(ModelNode[] modelNodes, WeightFunction function, Balancer balancer) {
this.function = function;
this.balancer = balancer;
this.modelNodes = modelNodes;
weights = new float[modelNodes.length];
}
/**
* Resets the sorter, recalculates the weights per node and sorts the
* nodes by weight, with minimal weight first.
*/
public void reset(String index, int from, int to) {
this.index = index;
for (int i = from; i < to; i++) {
weights[i] = weight(modelNodes[i]);
}
sort(from, to);
}
public void reset(String index) {
reset(index, 0, modelNodes.length);
}
public float weight(ModelNode node) {
return function.weight(balancer, node, index);
}
@Override
protected void swap(int i, int j) {
final ModelNode tmpNode = modelNodes[i];
modelNodes[i] = modelNodes[j];
modelNodes[j] = tmpNode;
final float tmpWeight = weights[i];
weights[i] = weights[j];
weights[j] = tmpWeight;
}
@Override
protected int compare(int i, int j) {
return Float.compare(weights[i], weights[j]);
}
@Override
protected void setPivot(int i) {
pivotWeight = weights[i];
}
@Override
protected int comparePivot(int j) {
return Float.compare(pivotWeight, weights[j]);
}
public float delta() {
return weights[weights.length - 1] - weights[0];
}
}
}
| {
"content_hash": "5660ac63d2768cb29b43971094721a0d",
"timestamp": "",
"source": "github",
"line_count": 1011,
"max_line_length": 229,
"avg_line_length": 49.00494559841741,
"alnum_prop": 0.5459793315033102,
"repo_name": "myelin/elasticsearch",
"id": "b1d78fa44d05fae597f6fc07c55ef37ece646aec",
"size": "50332",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "core/src/main/java/org/elasticsearch/cluster/routing/allocation/allocator/BalancedShardsAllocator.java",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "ANTLR",
"bytes": "9678"
},
{
"name": "Batchfile",
"bytes": "15897"
},
{
"name": "Emacs Lisp",
"bytes": "3341"
},
{
"name": "FreeMarker",
"bytes": "45"
},
{
"name": "Groovy",
"bytes": "249910"
},
{
"name": "HTML",
"bytes": "5595"
},
{
"name": "Java",
"bytes": "34902879"
},
{
"name": "Perl",
"bytes": "7116"
},
{
"name": "Python",
"bytes": "76106"
},
{
"name": "Shell",
"bytes": "102564"
}
],
"symlink_target": ""
} |
=head1 LICENSE
Copyright [1999-2014] Wellcome Trust Sanger Institute and the EMBL-European Bioinformatics Institute
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
=cut
package EnsEMBL::ORM::Component::DbFrontend;
### NAME: EnsEMBL::ORM::Component::DbFrontend;
### Base class for components that make up the Ensembl DbFrontend CRUD interface
### STATUS: Under Development
### DESCRIPTION:
### This module contains a lot of generic HTML/form generation code that is shared between DbFrontend CRUD pages.
use strict;
use warnings;
use Rose::DateTime::Util qw(parse_date format_date);
use EnsEMBL::ORM::Rose::Field;
use base qw(EnsEMBL::Web::Component);
use constant {
_JS_CLASS_DBF_RECORD => '_dbf_record',
_JS_CLASS_RESPONSE_ELEMENT => '_dbf_response',
_JS_CLASS_BUTTON => '_dbf_button',
_JS_CLASS_EDIT_BUTTON => '_dbf_edit',
_JS_CLASS_DELETE_BUTTON => '_dbf_delete',
_JS_CLASS_CANCEL_BUTTON => '_dbf_cancel',
_JS_CLASS_PREVIEW_FORM => '_dbf_preview',
_JS_CLASS_SAVE_FORM => '_dbf_save',
_JS_CLASS_ADD_FORM => '_dbf_add',
_JS_CLASS_DATASTRUCTURE => '_datastructure',
_JS_CLASS_LIST_TABLE => '_dbf_list',
_JS_CLASS_LIST_ROW_HANDLE => '_dbf_row_handle',
_JS_CLASS_DATATABLE => 'data_table no_col_toggle',
_FLAG_NO_CONTENT => '_dbf_no_content',
_FLAG_RECORD_BUTTONS => '_dbf_record_buttons'
};
sub _init {
my $self = shift;
$self->cacheable( 0 );
$self->ajaxable( 0 );
}
sub content {
## Returns content HTML
return shift->content_tree->render;
}
sub content_tree {
## Returns content html's dom tree
## Override in the child classes
my $self = shift;
my $object = $self->object;
return $self->dom->create_element('div', {
'class' => $object->content_css,
'children' => [
{'node_name' => 'h2', 'inner_HTML' => sprintf('No %s found', $object->record_name->{'plural'})},
{'node_name' => 'p', 'inner_HTML' => sprintf('No %s found in the database', $object->record_name->{'plural'}), 'flags' => $self->_FLAG_NO_CONTENT}
]
});
}
sub content_pagination {
## Generates HTML for pagination links
## @param Number of records being displayed on the page
## @return html string
return shift->content_pagination_tree(@_)->render;
}
sub content_pagination_tree {
## Generates and returns a DOM tree of pagination links
## @param Number of records being displayed on the page
## @return E::W::DOM::Node::Element::Div object
my ($self, $records_count) = @_;
my $object = $self->object;
my $hub = $self->hub;
my $page = $object->get_page_number;
my $page_count = $object->get_page_count;
my $count = $object->get_count;
my $offset = ($page - 1) * $object->pagination;
my $pagination = $self->dom->create_element('div', {'class' => 'dbf-pagination _dbf_pagination', 'flags' => 'pagination_div'});
my $links = $pagination->append_child($self->dom->create_element('div', {'class' => 'dbf-pagelinks', 'flags' => 'pagination_links'}));
$page < 1 and $page = 1 or $page > $page_count and $page = $page_count;
my $page_counter = $pagination->prepend_child('p', {
'class' => 'dbf-pagecount',
'flags' => 'page_counter',
'inner_HTML' => sprintf("Page %d of %d (displaying %d - %d of %d %s)", $page, $page_count, $offset + 1, $offset + $records_count, $count, $object->record_name->{$count == 1 ? 'singular' : 'plural'})
});
$links->append_child($self->dom->create_element('a', {
'href' => $hub->url({'page' => $page - 1 || 1}, undef, 1),
'inner_HTML' => '« Previous',
$page == 1 ?
('class' => 'disabled') : (),
}));
my $pages_needed = { map {$_ => 1} 1, 2, $page_count, $page_count - 1, $page, $page - 1, $page - 2, $page + 1, $page + 2 };
my $previous_num = 0;
for (sort {$a <=> $b} keys %$pages_needed) {
next if $_ <= 0 || $_ > $page_count;
for my $num ($_ - $previous_num > 4 ? ($_) : ($previous_num + 1 .. $_)) {
$num > $previous_num + 1 and $links->append_child($self->dom->create_element('span', {'inner_HTML' => '…'}));
$links->append_child($self->dom->create_element('a', {
'href' => $hub->url({'page' => $num}, undef, 1),
'inner_HTML' => $num,
$page == $num ?
('class' => 'selected') : ()
}));
$previous_num = $num;
}
$previous_num = $_;
}
$links->append_child($self->dom->create_element('a', {
'href' => $hub->url({'page' => $page_count - ($page_count - $page || 1) + 1}, undef, 1),
'inner_HTML' => 'Next »',
$page == $page_count ?
('class' => 'disabled') : (),
}));
return $pagination;
}
sub unpack_rose_object {
## Converts a rose object, it's columns and relationships into a data structure that can easily be used to display frontend
## @param Rose object to be unpacked
## @param GET param values to override any value in the object field
## @return ArrayRef if E::ORM::Rose::Field objects
my ($self, $record, $url_params) = @_;
my $object = $self->object;
my $manager = $object->manager_class;
$record ||= $manager->create_empty_object;
my $meta = $record->meta;
my $fields = $object->get_fields;
my $relations = { map {$_->name => $_ } $meta->relationships };
my $columns = { map {$_->name => $_ } $meta->columns, $meta->virtual_columns };
my $unpacked = [];
while (my $field_name = shift @$fields) {
my $field = shift @$fields; # already a hashref with keys that should not be modified (except 'value' if its undef) - keys as accepted by Form->add_field method
my $value = exists $url_params->{$field_name} ? $url_params->{$field_name} : $record->field_value($field_name);
$value = $field->{'value'} unless defined $value;
$field->{'value'} = $value;
$field->{'name'} ||= $field_name;
my $select = $field->{'type'} && $field->{'type'} =~ /^(dropdown|checklist|radiolist)$/i ? 1 : 0;
## if this field is a relationship
if (exists $relations->{$field_name}) {
my $relationship = $relations->{$field_name};
my $related_object_meta = $relationship->can('class') ? $relationship->class->meta : $relationship->map_class->meta->relationship($relationship->name)->class->meta;
my $title_column = $related_object_meta->column($related_object_meta->title_column);
$field->{'is_datastructure'} = $title_column && $title_column->type eq 'datastructure';
$field->{'value_type'} = $relationship->type;
## get lookup if type is either 'dropdown' or 'checklist' or 'radiolist'
if ($select) {
$field->{'value'} = [];
$field->{'multiple'} = $relationship->is_singular ? 0 : 1;
$field->{'lookup'} = $manager->get_lookup($related_object_meta->class);
$field->{'selected'} = { map {
!ref $_
? exists $field->{'lookup'}{$_}
? ($_ => $field->{'lookup'}{$_})
: ()
: ($_->get_primary_key_value => $_->get_title)
} (ref $value eq 'ARRAY'
? ( $field->{'multiple'}
? @$value
: shift @$value
) : $value
) } if $value;
}
}
## if this field is a column
elsif (exists $columns->{$field_name}) {
my $column = $columns->{$field_name};
$field->{'value_type'} = 'noedit' if $column->type ne 'virtual' && $column->is_primary_key_member; #force readonly primary key
$field->{'is_datastructure'} = $column->type eq 'datastructure';
$field->{'is_column'} = 1;
if (($field->{'value_type'} = $column->type) =~ /^(enum|set)$/ || $select) {
$field->{'value'} = [];
$field->{'lookup'} = {};
$field->{'selected'} = {};
$field->{'multiple'} = $1 eq 'set' ? 1 : 0;
$value = defined $value ? { map {$_ => 1} (ref $value ? ($field->{'multiple'} ? @$value : shift @$value) : $value) } : {};
for (@{delete $field->{'values'} || ($column->can('values') ? $column->values : [])}) {
my $label;
if (ref $_) {
$label = $_->{'caption'};
$_ = $_->{'value'};
}
else {
($label = ucfirst $_) =~ s/_/ /g;
}
$field->{'lookup'}{$_} = $label;
$field->{'selected'}{$_} = $label if exists $value->{$_};
}
} else {
$field->{'value'} = shift @$value if ref $value eq 'ARRAY';
}
}
## if any external relation
else {
$field->{'value_type'} = 'one to one';
$field->{'selected'} = {$value->get_primary_key_value => $value->get_title} if $value;
}
push @$unpacked, EnsEMBL::ORM::Rose::Field->new($field);
}
return $unpacked;
}
sub print_datetime {
## Prints DateTime as a readable string
return format_date(parse_date($_[1]), "%b %e, %Y at %H:%M");
}
1; | {
"content_hash": "e61f512b5ad212d18b22cc21b421e0e1",
"timestamp": "",
"source": "github",
"line_count": 253,
"max_line_length": 204,
"avg_line_length": 37.76679841897233,
"alnum_prop": 0.5621140763997907,
"repo_name": "andrewyatz/public-plugins",
"id": "0b105d4321e7808771a9520595d3b584a71da619",
"size": "9555",
"binary": false,
"copies": "1",
"ref": "refs/heads/release/75",
"path": "orm/modules/EnsEMBL/ORM/Component/DbFrontend.pm",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "C",
"bytes": "241227"
},
{
"name": "C++",
"bytes": "517"
},
{
"name": "CSS",
"bytes": "111087"
},
{
"name": "CoffeeScript",
"bytes": "160369"
},
{
"name": "D",
"bytes": "9430"
},
{
"name": "JavaScript",
"bytes": "331919"
},
{
"name": "Perl",
"bytes": "981782"
},
{
"name": "Shell",
"bytes": "1236"
}
],
"symlink_target": ""
} |
package com.sababado.android.converter;
import org.apache.commons.io.FileUtils;
import java.io.File;
import java.io.FileFilter;
import java.io.IOException;
import java.io.InputStream;
/**
* Created by Robert on 8/24/13.
*/
public class Utils {
private static final String POST_FIX_BACKUP = "_apc_backup";
/**
* Types of dependencies.
*/
public enum DependencyType {
compile, instrumentTestCompile
}
/**
* Helper method to copy a file from resources to an external location.
*
* @param resourcePath Path to the resource to copy
* @param destPath Path to the file to end up.
* @return True if the operation is successful, false if there is an error.
*/
public static boolean copyFileFromResourcesToFile(final String resourcePath, final String destPath) {
System.out.println("Looking for resource: " + resourcePath);
final InputStream is = ClassLoader.getSystemResourceAsStream(resourcePath);
final File fileDest = new File(destPath);
try {
FileUtils.copyInputStreamToFile(is, fileDest);
} catch (IOException e) {
return false;
}
return true;
}
/**
* Get a directory with a given path.
*
* @param path Path to get a directory by.
* @param createIfDoesntExist True to create the directory if it doesn't exist, false to not.
* @return A non null File object, or null if the path doesn't exist or it isn't a directory.
*/
public static File getDirectory(final String path, final boolean createIfDoesntExist) {
assert (path != null);
File f = new File(path);
if (!f.exists() && !createIfDoesntExist) {
System.out.println("!! \"" + path + "\" does not exist.");
return null;
} else if (createIfDoesntExist) {
try {
FileUtils.forceMkdir(f);
} catch (IOException e) {
System.out.println("!! Could not make directory: " + path);
return null;
}
} else if (!f.isDirectory()) {
if (!createIfDoesntExist) {
//return if it doesn't exist or can't create it.
System.out.println("!! \"" + path + "\" is not a directory.");
return null;
}
}
return f;
}
/**
* Make a backup of a given directory.
*
* @param sourceDir Directory to make a backup of.
* @return Returns the directory if it was successfully backed-up. Null if there was an error.
*/
public static File makeBackup(final File sourceDir) {
assert (sourceDir != null);
//make a backup.
final File copyDir = getBackupDirectory(sourceDir, POST_FIX_BACKUP);
try {
FileUtils.copyDirectory(sourceDir, copyDir);
} catch (IOException e) {
System.out.println("Could not make a backup");
e.printStackTrace();
return null;
}
return copyDir;
}
/**
* Get the directory to backup to. The directory will be next to the source directory and have the post fix concatenated to it.
*
* @param sourceDir Directory backup from.
* @param destPostFix Post fix to append to the directory name.
* @return Return the directory that should be used as a backup, null if there is an error.
*/
public static File getBackupDirectory(final File sourceDir, String destPostFix) {
assert (sourceDir != null);
assert (sourceDir.isDirectory());
if (destPostFix == null)
destPostFix = "";
//get copy directory
String copyDirPath = sourceDir.getPath().concat(destPostFix);
final String baseCopyDirPath = copyDirPath;
File copyDir = new File(copyDirPath);
int counter = 1;
//don't use one that already exists.
while (copyDir.exists()) {
copyDir = new File(baseCopyDirPath + String.valueOf(counter));
System.out.println("New backup destination: " + copyDir.getPath());
counter++;
}
//make the directory
try {
FileUtils.forceMkdir(copyDir);
} catch (IOException e) {
System.out.println("Could not make the directory: " + copyDirPath);
return null;
}
return copyDir;
}
/**
* Get an argument from the args array.
*
* @param args Arguments to get from
* @param defaultIndex Default index in the case of no preceding optional parameters
* @param indexOffset Index offset in the case of any preceding optional parameters.
* @return An argument as a string.
* @throws IndexOutOfBoundsException Thrown if there indices are off.
*/
public static String getArgument(final String[] args, final int defaultIndex, final int indexOffset) throws IndexOutOfBoundsException {
return args[defaultIndex + indexOffset];
}
/**
* File filer. Filters any IDE specific files (Eclipse, or Intellij) as well as environment local files.
*/
public static final FileFilter IDE_FILTER = new FileFilter() {
@Override
public boolean accept(File pathname) {
final String name = pathname.getName();
if (name.equals(".classpath") ||
name.equals(".project") ||
name.equals("project.properties") ||
name.equals("local.properties") ||
name.equals(".gradle") ||
name.equals(".iml") ||
name.equals("bin") ||
name.equals("gen")) {
return false;
}
return true;
}
};
}
| {
"content_hash": "151cc435c72594cc53a3e062207e6af3",
"timestamp": "",
"source": "github",
"line_count": 160,
"max_line_length": 139,
"avg_line_length": 36.2875,
"alnum_prop": 0.5907681708577334,
"repo_name": "sababado/AndroidProjectConverter",
"id": "d6d98faa2f4184b8d3f1177f256d3ae471ae152b",
"size": "5806",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "src/main/java/com/sababado/android/converter/Utils.java",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "Groovy",
"bytes": "683"
},
{
"name": "Java",
"bytes": "41452"
},
{
"name": "Shell",
"bytes": "2314"
}
],
"symlink_target": ""
} |
#ifndef _VM_OBJECT_H_
#define _VM_OBJECT_H_
#include "lib/primitives/sys/tree.h"
#include "pmap.h"
#include "vm.h"
#ifdef __cplusplus
extern "C" {
#endif
/* At the moment assume object is owned by only one vm_map */
typedef struct vm_object {
TAILQ_HEAD(, vm_page) list;
RB_HEAD(vm_object_tree, vm_page) tree;
size_t size;
size_t npages;
pager_t *pgr;
} vm_object_t;
void vm_object_init();
/* TODO in future this function will need to be parametrized by type */
vm_object_t *vm_object_alloc();
void vm_object_free(vm_object_t *obj);
int vm_object_add_page(vm_object_t *obj, vm_page_t *pg);
void vm_object_remove_page(vm_object_t *obj, vm_page_t *pg);
vm_page_t *vm_object_find_page(vm_object_t *obj, vm_addr_t offset);
void vm_map_object_dump(vm_object_t *obj);
#ifdef __cplusplus
}
#endif
#endif /* _VM_OBJECT_H_ */
| {
"content_hash": "5c954684294dbd05150a24a18b71759c",
"timestamp": "",
"source": "github",
"line_count": 36,
"max_line_length": 71,
"avg_line_length": 23.47222222222222,
"alnum_prop": 0.6745562130177515,
"repo_name": "goniz/gzOS",
"id": "9b9d2f76000db39ce23f5e9245ba56e195e6b332",
"size": "2164",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "kernel/lib/mm/vm_object.h",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "Assembly",
"bytes": "20288"
},
{
"name": "C",
"bytes": "4061898"
},
{
"name": "C++",
"bytes": "478005"
},
{
"name": "CMake",
"bytes": "20369"
},
{
"name": "GDB",
"bytes": "233"
},
{
"name": "Makefile",
"bytes": "1686"
},
{
"name": "Shell",
"bytes": "3033"
}
],
"symlink_target": ""
} |
<?xml version="1.0" ?><!DOCTYPE TS><TS language="fr_FR" version="2.0">
<context>
<name>AccountManager</name>
<message>
<location filename="../src/account-mgr.cpp" line="36"/>
<source>failed to open account database</source>
<translation>Impossible d'ouvrir la base de données des comptes</translation>
</message>
</context>
<context>
<name>AccountSettingsDialog</name>
<message>
<location filename="../src/ui/account-settings-dialog.cpp" line="18"/>
<source>Account Settings</source>
<translation>Paramètres du compte</translation>
</message>
<message>
<location filename="../src/ui/account-settings-dialog.cpp" line="53"/>
<source>Please enter the server address</source>
<translation>Entrez l'adresse du serveur</translation>
</message>
<message>
<location filename="../src/ui/account-settings-dialog.cpp" line="57"/>
<location filename="../src/ui/account-settings-dialog.cpp" line="63"/>
<source>%1 is not a valid server address</source>
<translation>%1 n'est pas une adresse de serveur valide</translation>
</message>
<message>
<location filename="../src/ui/account-settings-dialog.cpp" line="83"/>
<source>Failed to save account information</source>
<translation>Échec de la sauvegarde des informations du compte</translation>
</message>
<message>
<location filename="../src/ui/account-settings-dialog.cpp" line="89"/>
<source>Failed to save the changes: %1</source>
<translation>Échec de la sauvegarde des changements: %1</translation>
</message>
<message>
<location filename="../src/ui/account-settings-dialog.cpp" line="94"/>
<source>Successfully updated current account information</source>
<translation>Information du compte mise à jour avec succès</translation>
</message>
<message>
<location filename="../ui_account-settings-dialog.h" line="107"/>
<source>Dialog</source>
<translation>Dialogue</translation>
</message>
<message>
<location filename="../ui_account-settings-dialog.h" line="108"/>
<source>Server Address</source>
<translation>Adresse du serveur</translation>
</message>
<message>
<location filename="../ui_account-settings-dialog.h" line="109"/>
<source>Email</source>
<translation>Courriel</translation>
</message>
<message>
<location filename="../ui_account-settings-dialog.h" line="110"/>
<source>OK</source>
<translation>OK</translation>
</message>
<message>
<location filename="../ui_account-settings-dialog.h" line="111"/>
<source>Cancel</source>
<translation>Annuler</translation>
</message>
</context>
<context>
<name>AccountView</name>
<message>
<location filename="../src/ui/account-view.cpp" line="55"/>
<source>Are you sure to remove this account?<br><b>Warning: All libraries of this account would be unsynced!</b></source>
<translation>Êtes-vous sûr de vouloir supprimer ce compte ?<br><b>Avertissement : Toutes les bibliothèques de ce compte seront désynchronisées</b ></translation>
</message>
<message>
<location filename="../src/ui/account-view.cpp" line="65"/>
<source>Failed to unsync libraries of this account: %1</source>
<translation>Impossible de désynchroniser les bibliothèques de ce compte:%1</translation>
</message>
<message>
<location filename="../src/ui/account-view.cpp" line="87"/>
<source>click to open the website</source>
<translation>cliquez pour ouvrir le site web</translation>
</message>
<message>
<location filename="../src/ui/account-view.cpp" line="97"/>
<source>No account</source>
<translation>Pas de compte</translation>
</message>
<message>
<location filename="../src/ui/account-view.cpp" line="129"/>
<source>Account settings</source>
<translation>Paramètres du compte</translation>
</message>
<message>
<location filename="../src/ui/account-view.cpp" line="136"/>
<source>Add an account</source>
<translation>Ajouter un compte</translation>
</message>
<message>
<location filename="../src/ui/account-view.cpp" line="143"/>
<source>Delete this account</source>
<translation>Supprimer ce compte</translation>
</message>
<message>
<location filename="../ui_account-view.h" line="82"/>
<source>Form</source>
<translation>Formulaire</translation>
</message>
<message>
<location filename="../ui_account-view.h" line="83"/>
<source>Account</source>
<translation>Compte</translation>
</message>
<message>
<location filename="../ui_account-view.h" line="84"/>
<source>email</source>
<translation>courriel</translation>
</message>
<message>
<location filename="../ui_account-view.h" line="85"/>
<source>server</source>
<translation>serveur</translation>
</message>
</context>
<context>
<name>ActivitiesTab</name>
<message>
<location filename="../src/ui/activities-tab.cpp" line="105"/>
<source>More</source>
<translation>Plus</translation>
</message>
<message>
<location filename="../src/ui/activities-tab.cpp" line="150"/>
<source>File Activities are only supported in Seafile Server Professional Edition.</source>
<translation>Les activités de Fichier sont seulement supportées dans la version professionnelle du Serveur Seafile.</translation>
</message>
<message>
<location filename="../src/ui/activities-tab.cpp" line="152"/>
<source>retry</source>
<translation>réessayer </translation>
</message>
<message>
<location filename="../src/ui/activities-tab.cpp" line="153"/>
<source>Failed to get actvities information. Please %1</source>
<translation>Impossible de récupérer les informations des activités. Veuillez %1</translation>
</message>
</context>
<context>
<name>AvatarService</name>
<message>
<location filename="../src/avatar-service.cpp" line="141"/>
<source>Failed to create avatars folder</source>
<translation>Impossible de créer le dossier des avatars</translation>
</message>
</context>
<context>
<name>CloneTasksDialog</name>
<message>
<location filename="../src/ui/clone-tasks-dialog.cpp" line="30"/>
<source>Download tasks</source>
<translation>Tâches de téléchargement</translation>
</message>
<message>
<location filename="../src/ui/clone-tasks-dialog.cpp" line="48"/>
<source>remove all successful tasks</source>
<translation>supprimer toutes les tâches terminées avec succès</translation>
</message>
<message>
<location filename="../src/ui/clone-tasks-dialog.cpp" line="79"/>
<source>No download tasks right now.</source>
<translation>Pas de tâches de téléchargement en cours.</translation>
</message>
<message>
<location filename="../ui_clone-tasks-dialog.h" line="69"/>
<source>Dialog</source>
<translation>Dialogue</translation>
</message>
<message>
<location filename="../ui_clone-tasks-dialog.h" line="70"/>
<source>Clear</source>
<translation>Vider</translation>
</message>
<message>
<location filename="../ui_clone-tasks-dialog.h" line="71"/>
<source>Close</source>
<translation>Fermer</translation>
</message>
</context>
<context>
<name>CloneTasksTableModel</name>
<message>
<location filename="../src/ui/clone-tasks-table-model.cpp" line="110"/>
<source>Library</source>
<translation>Bibliothèques</translation>
</message>
<message>
<location filename="../src/ui/clone-tasks-table-model.cpp" line="117"/>
<source>Path</source>
<translation>Répertoire</translation>
</message>
</context>
<context>
<name>CloneTasksTableView</name>
<message>
<location filename="../src/ui/clone-tasks-table-view.cpp" line="72"/>
<source>Cancel this task</source>
<translation>Annuler cette tâche</translation>
</message>
<message>
<location filename="../src/ui/clone-tasks-table-view.cpp" line="74"/>
<source>cancel this task</source>
<translation>annuler cette tâche</translation>
</message>
<message>
<location filename="../src/ui/clone-tasks-table-view.cpp" line="79"/>
<location filename="../src/ui/clone-tasks-table-view.cpp" line="81"/>
<source>Remove this task</source>
<translation>Supprimer cette tâche</translation>
</message>
<message>
<location filename="../src/ui/clone-tasks-table-view.cpp" line="93"/>
<source>Failed to cancel this task:
%1</source>
<translation>Impossible d'annuler cette tâche:
%1</translation>
</message>
<message>
<location filename="../src/ui/clone-tasks-table-view.cpp" line="104"/>
<source>Failed to remove this task:
%1</source>
<translation>Impossible de supprimer cette tâche:
%1</translation>
</message>
</context>
<context>
<name>CloudView</name>
<message>
<location filename="../src/ui/cloud-view.cpp" line="106"/>
<source>Minimize</source>
<translation>Minimiser</translation>
</message>
<message>
<location filename="../src/ui/cloud-view.cpp" line="111"/>
<source>Close</source>
<translation>Fermer</translation>
</message>
<message>
<location filename="../src/ui/cloud-view.cpp" line="133"/>
<source>Libraries</source>
<translation>Bibliothèques</translation>
</message>
<message>
<location filename="../src/ui/cloud-view.cpp" line="136"/>
<source>Starred</source>
<translation>Favoris</translation>
</message>
<message>
<location filename="../src/ui/cloud-view.cpp" line="151"/>
<source>Activities</source>
<translation>Activités</translation>
</message>
<message>
<location filename="../src/ui/cloud-view.cpp" line="181"/>
<source>current download rate</source>
<translation>vitesse actuelle de téléchargement</translation>
</message>
<message>
<location filename="../src/ui/cloud-view.cpp" line="187"/>
<source>current upload rate</source>
<translation>vitesse actuelle d'envoi</translation>
</message>
<message>
<location filename="../src/ui/cloud-view.cpp" line="192"/>
<source>Please Choose a folder to sync</source>
<translation>Veuillez choisir un dossier à synchroniser</translation>
</message>
<message>
<location filename="../src/ui/cloud-view.cpp" line="319"/>
<source>no server connected</source>
<translation>pas de serveur connecté</translation>
</message>
<message>
<location filename="../src/ui/cloud-view.cpp" line="317"/>
<source>all servers connected</source>
<translation>tous les serveurs sont connectés</translation>
</message>
<message>
<location filename="../src/ui/cloud-view.cpp" line="321"/>
<source>some servers not connected</source>
<translation>des serveurs ne sont pas connectés</translation>
</message>
<message>
<location filename="../src/ui/cloud-view.cpp" line="340"/>
<location filename="../src/ui/cloud-view.cpp" line="341"/>
<source>%1 kB/s</source>
<translation>%1 Ko/s</translation>
</message>
<message>
<location filename="../src/ui/cloud-view.cpp" line="389"/>
<source>Refresh</source>
<translation>Actualiser</translation>
</message>
<message>
<location filename="../ui_cloud-view.h" line="231"/>
<source>Form</source>
<translation>Formulaire</translation>
</message>
<message>
<location filename="../ui_cloud-view.h" line="232"/>
<source>logo</source>
<translation>logo</translation>
</message>
<message>
<location filename="../ui_cloud-view.h" line="233"/>
<source>Seafile</source>
<translation>Seafile</translation>
</message>
<message>
<location filename="../ui_cloud-view.h" line="234"/>
<source>minimize</source>
<translation>minimiser</translation>
</message>
<message>
<location filename="../ui_cloud-view.h" line="235"/>
<source>close</source>
<translation>fermer</translation>
</message>
<message>
<location filename="../ui_cloud-view.h" line="238"/>
<source>...</source>
<translation>...</translation>
</message>
<message>
<location filename="../ui_cloud-view.h" line="236"/>
<source>Select</source>
<translation>Sélectionnez </translation>
</message>
<message>
<location filename="../ui_cloud-view.h" line="237"/>
<source>or Drop Folder to Sync</source>
<translation>ou déposez un dossier à synchroniser</translation>
</message>
<message>
<location filename="../ui_cloud-view.h" line="239"/>
<source>download rate</source>
<translation>vitesse de téléchargement</translation>
</message>
<message>
<location filename="../ui_cloud-view.h" line="240"/>
<source>downarrow</source>
<translation>flèchebas</translation>
</message>
<message>
<location filename="../ui_cloud-view.h" line="241"/>
<source>upload rate</source>
<translation>vitesse d'envoi</translation>
</message>
<message>
<location filename="../ui_cloud-view.h" line="242"/>
<source>uparrow</source>
<translation>flèchehaut</translation>
</message>
</context>
<context>
<name>Configurator</name>
<message>
<location filename="../src/configurator.cpp" line="73"/>
<source>Error when creating ccnet configuration</source>
<translation>Erreur lors de la création de la configuration de ccnet</translation>
</message>
<message>
<location filename="../src/configurator.cpp" line="187"/>
<source>failed to read %1</source>
<translation>impossible de lire %1</translation>
</message>
<message>
<location filename="../src/configurator.cpp" line="232"/>
<source>%1 Default Library</source>
<translation>Bibliothèque par défaut de %1</translation>
</message>
</context>
<context>
<name>CreateRepoDialog</name>
<message>
<location filename="../src/ui/create-repo-dialog.cpp" line="21"/>
<source>Create a library</source>
<translation>Créer une bibliothèque</translation>
</message>
<message>
<location filename="../src/ui/create-repo-dialog.cpp" line="44"/>
<source>Please choose a directory</source>
<translation>Veuillez choisir un répertoire</translation>
</message>
<message>
<location filename="../src/ui/create-repo-dialog.cpp" line="76"/>
<source>Creating...</source>
<translation>Création...</translation>
</message>
<message>
<location filename="../src/ui/create-repo-dialog.cpp" line="103"/>
<source>Please choose the directory to sync</source>
<translation>Veuillez choisir le répertoire à synchroniser</translation>
</message>
<message>
<location filename="../src/ui/create-repo-dialog.cpp" line="107"/>
<source>The folder %1 does not exist</source>
<translation>Le dossier %1 n'existe pas</translation>
</message>
<message>
<location filename="../src/ui/create-repo-dialog.cpp" line="112"/>
<source>Please enter the name</source>
<translation>Veuillez entrer un nom</translation>
</message>
<message>
<location filename="../src/ui/create-repo-dialog.cpp" line="117"/>
<source>Please enter the description</source>
<translation>Veuillez entrer une description</translation>
</message>
<message>
<location filename="../src/ui/create-repo-dialog.cpp" line="124"/>
<source>Please enter the password</source>
<translation>Veuillez entrer un mot de passe</translation>
</message>
<message>
<location filename="../src/ui/create-repo-dialog.cpp" line="131"/>
<source>Passwords don't match</source>
<translation>Les mots de passe ne correspondent pas</translation>
</message>
<message>
<location filename="../src/ui/create-repo-dialog.cpp" line="142"/>
<source>Unknown error</source>
<translation>Erreur inconnue</translation>
</message>
<message>
<location filename="../src/ui/create-repo-dialog.cpp" line="177"/>
<source>Failed to add download task:
%1</source>
<translation>Impossible d'ajouter la tâche de téléchargement:
%1</translation>
</message>
<message>
<location filename="../src/ui/create-repo-dialog.cpp" line="189"/>
<source>Failed to create library on the server:
%1</source>
<translation>Impossible de créer la bibliothèque sur le serveur:
%1</translation>
</message>
<message>
<location filename="../ui_create-repo-dialog.h" line="194"/>
<source>Dialog</source>
<translation>Dialogue</translation>
</message>
<message>
<location filename="../ui_create-repo-dialog.h" line="195"/>
<source>Path:</source>
<translation>Répertoire :</translation>
</message>
<message>
<location filename="../ui_create-repo-dialog.h" line="196"/>
<source>Choose</source>
<translation>Choisir</translation>
</message>
<message>
<location filename="../ui_create-repo-dialog.h" line="197"/>
<source>Name:</source>
<translation>Nom :</translation>
</message>
<message>
<location filename="../ui_create-repo-dialog.h" line="198"/>
<source>Description:</source>
<translation>Description :</translation>
</message>
<message>
<location filename="../ui_create-repo-dialog.h" line="199"/>
<source>encrypted</source>
<translation>chiffré</translation>
</message>
<message>
<location filename="../ui_create-repo-dialog.h" line="200"/>
<source>Password:</source>
<translation>Mot de passe :</translation>
</message>
<message>
<location filename="../ui_create-repo-dialog.h" line="201"/>
<source>Password Again:</source>
<translation>Vérification du mot de passe :</translation>
</message>
<message>
<location filename="../ui_create-repo-dialog.h" line="202"/>
<source>status text</source>
<translation>texte de l'état</translation>
</message>
<message>
<location filename="../ui_create-repo-dialog.h" line="203"/>
<source>OK</source>
<translation>OK</translation>
</message>
<message>
<location filename="../ui_create-repo-dialog.h" line="204"/>
<source>Cancel</source>
<translation>Annuler</translation>
</message>
</context>
<context>
<name>DaemonManager</name>
<message>
<location filename="../src/daemon-mgr.cpp" line="59"/>
<source>failed to load ccnet config dir %1</source>
<translation>impossible de charger le répertoire de configuration de ccnet %1 </translation>
</message>
</context>
<context>
<name>DownloadRepoDialog</name>
<message>
<location filename="../src/ui/download-repo-dialog.cpp" line="49"/>
<source>Choose a folder</source>
<translation>Choisissez un dossier</translation>
</message>
<message>
<location filename="../src/ui/download-repo-dialog.cpp" line="106"/>
<source>Create a new sync folder at:</source>
<translation>Créer un nouveau dossier synchronisé à :</translation>
</message>
<message>
<location filename="../src/ui/download-repo-dialog.cpp" line="146"/>
<source>Please choose a directory</source>
<translation>Veuillez choisir un répertoire</translation>
</message>
<message>
<location filename="../src/ui/download-repo-dialog.cpp" line="177"/>
<source>Please choose the folder to sync</source>
<translation>Veuillez choisir le dossier à synchroniser</translation>
</message>
<message>
<location filename="../src/ui/download-repo-dialog.cpp" line="184"/>
<source>The folder does not exist</source>
<translation>Le dossier n'existe pas</translation>
</message>
<message>
<location filename="../src/ui/download-repo-dialog.cpp" line="192"/>
<source>Please enter the password</source>
<translation>Veuillez entrer le mot de passe</translation>
</message>
<message>
<location filename="../src/ui/download-repo-dialog.cpp" line="43"/>
<source>Sync library "%1"</source>
<translation>Synchroniser la bibliothèque "%1"</translation>
</message>
<message>
<location filename="../src/ui/download-repo-dialog.cpp" line="101"/>
<source>or</source>
<translation>ou</translation>
</message>
<message>
<location filename="../src/ui/download-repo-dialog.cpp" line="103"/>
<source>sync with an existing folder</source>
<translation>synchroniser avec un dossier existant</translation>
</message>
<message>
<location filename="../src/ui/download-repo-dialog.cpp" line="113"/>
<source>create a new sync folder</source>
<translation>créer un nouveau dossier synchronisé</translation>
</message>
<message>
<location filename="../src/ui/download-repo-dialog.cpp" line="116"/>
<source>Sync with this existing folder:</source>
<translation>Synchroniser avec un dossier existant :</translation>
</message>
<message>
<location filename="../src/ui/download-repo-dialog.cpp" line="242"/>
<source>Failed to add download task:
%1</source>
<translation>Impossible d'ajouter la tâche de téléchargement:
%1</translation>
</message>
<message>
<location filename="../src/ui/download-repo-dialog.cpp" line="253"/>
<source>Failed to get repo download information:
%1</source>
<translation>Impossible de récupérer les informations du repo :
%1</translation>
</message>
<message>
<location filename="../ui_download-repo-dialog.h" line="224"/>
<source>Download Library</source>
<translation>Télécharger la bibliothèque</translation>
</message>
<message>
<location filename="../ui_download-repo-dialog.h" line="225"/>
<source>choose...</source>
<translation>choisir...</translation>
</message>
<message>
<location filename="../ui_download-repo-dialog.h" line="226"/>
<source>Password for this library:</source>
<translation>Mot de passe de cette bibliothèque :</translation>
</message>
<message>
<location filename="../ui_download-repo-dialog.h" line="227"/>
<source>OK</source>
<translation>OK</translation>
</message>
<message>
<location filename="../ui_download-repo-dialog.h" line="228"/>
<source>Cancel</source>
<translation>Annuler</translation>
</message>
</context>
<context>
<name>EventDetailsDialog</name>
<message>
<location filename="../src/ui/event-details-dialog.cpp" line="30"/>
<source>Modification Details</source>
<translation>Détail des modifications</translation>
</message>
</context>
<context>
<name>EventDetailsTreeModel</name>
<message>
<location filename="../src/ui/event-details-tree.cpp" line="110"/>
<source>Added files</source>
<translation>Fichiers ajoutés</translation>
</message>
<message>
<location filename="../src/ui/event-details-tree.cpp" line="111"/>
<source>Deleted files</source>
<translation>Fichiers supprimés</translation>
</message>
<message>
<location filename="../src/ui/event-details-tree.cpp" line="112"/>
<source>Modified files</source>
<translation>Fichiers modifiés</translation>
</message>
<message>
<location filename="../src/ui/event-details-tree.cpp" line="114"/>
<source>Added folders</source>
<translation>Dossiers ajoutés</translation>
</message>
<message>
<location filename="../src/ui/event-details-tree.cpp" line="115"/>
<source>Deleted folders</source>
<translation>Dossiers supprimés</translation>
</message>
<message>
<location filename="../src/ui/event-details-tree.cpp" line="122"/>
<source>Renamed files</source>
<translation>Fichiers renommés</translation>
</message>
</context>
<context>
<name>FileBrowserDialog</name>
<message>
<location filename="../src/filebrowser/file-browser-dialog.cpp" line="71"/>
<source>Cloud File Browser</source>
<translation>Navigateur de fichiers</translation>
</message>
<message>
<location filename="../src/filebrowser/file-browser-dialog.cpp" line="162"/>
<source>Back</source>
<translation>Retour</translation>
</message>
<message>
<location filename="../src/filebrowser/file-browser-dialog.cpp" line="168"/>
<source>Forward</source>
<translation>Avancer</translation>
</message>
<message>
<location filename="../src/filebrowser/file-browser-dialog.cpp" line="174"/>
<source>Home</source>
<translation>Dossier personnel</translation>
</message>
<message>
<location filename="../src/filebrowser/file-browser-dialog.cpp" line="226"/>
<source>You don't have permission to upload files to this library</source>
<translation>Vous n'avez pas les droits pour téléverser des fichiers dans cette bibliothèque</translation>
</message>
<message>
<location filename="../src/filebrowser/file-browser-dialog.cpp" line="213"/>
<location filename="../src/filebrowser/file-browser-dialog.cpp" line="309"/>
<source>Create a folder</source>
<translation>Créer un dossier</translation>
</message>
<message>
<location filename="../src/filebrowser/file-browser-dialog.cpp" line="208"/>
<source>Upload a file</source>
<translation>Envoyer un fichier</translation>
</message>
<message>
<location filename="../src/filebrowser/file-browser-dialog.cpp" line="310"/>
<source>Folder name</source>
<translation>Nom du dossier</translation>
</message>
<message>
<location filename="../src/filebrowser/file-browser-dialog.cpp" line="318"/>
<source>Invalid folder name!</source>
<translation>Nom de dossier invalide</translation>
</message>
<message>
<location filename="../src/filebrowser/file-browser-dialog.cpp" line="324"/>
<source>The name "%1" is already taken.</source>
<translation>Le nom "%1" est déjà utilisé.</translation>
</message>
<message>
<location filename="../src/filebrowser/file-browser-dialog.cpp" line="340"/>
<source>retry</source>
<translation>réessayer </translation>
</message>
<message>
<location filename="../src/filebrowser/file-browser-dialog.cpp" line="341"/>
<source>Failed to get files information<br/>Please %1</source>
<translation>Impossible de récupérer les informations des fichiers <br/>Veuillez %1</translation>
</message>
<message>
<location filename="../src/filebrowser/file-browser-dialog.cpp" line="451"/>
<source>Feature not supported</source>
<translation>Fonctionnalité non supportée</translation>
</message>
<message>
<location filename="../src/filebrowser/file-browser-dialog.cpp" line="484"/>
<source>File %1 already exists.<br/>Do you like to overwrite it?<br/><small>(Choose No to upload using an alternative name).</small></source>
<translation>Le fichier %1 existe déja.<br/>Voulez vous le remplacer?<br/><small>(Choisissez Non pour l'enregistrer avec un autre nom).</small></translation>
</message>
<message>
<location filename="../src/filebrowser/file-browser-dialog.cpp" line="518"/>
<source>Failed to download file: %1</source>
<translation>Échec du téléchargement du fichier: %1</translation>
</message>
<message>
<location filename="../src/filebrowser/file-browser-dialog.cpp" line="563"/>
<source>Failed to upload file: %1</source>
<translation>Échec du téléversement du fichier: %1</translation>
</message>
<message>
<location filename="../src/filebrowser/file-browser-dialog.cpp" line="630"/>
<source>Select a file to upload</source>
<translation>Séléctionner un fichier à envoyer</translation>
</message>
<message>
<location filename="../src/filebrowser/file-browser-dialog.cpp" line="641"/>
<source>Unable to create cache folder</source>
<translation>Impossible de créer le dossier de cache</translation>
</message>
<message>
<location filename="../src/filebrowser/file-browser-dialog.cpp" line="643"/>
<source>Unable to open cache folder</source>
<translation>Impossible d'ouvrir le dossier de cache</translation>
</message>
<message>
<location filename="../src/filebrowser/file-browser-dialog.cpp" line="663"/>
<source>Rename</source>
<translation>Renommer</translation>
</message>
<message>
<location filename="../src/filebrowser/file-browser-dialog.cpp" line="685"/>
<source>Do you really want to delete file "%1"?</source>
<translation>Souhaitez vous vraiment supprimer le fichier "%1" ?</translation>
</message>
<message>
<location filename="../src/filebrowser/file-browser-dialog.cpp" line="686"/>
<source>Do you really want to delete folder "%1"?</source>
<translation>Souhaitez vous vraiment supprimer le dossier "%1" ?</translation>
</message>
<message>
<location filename="../src/filebrowser/file-browser-dialog.cpp" line="693"/>
<source>Do you really want to delete these items</source>
<translation>Voulez-vous vraiment effacer ces éléments</translation>
</message>
<message>
<location filename="../src/filebrowser/file-browser-dialog.cpp" line="724"/>
<source>Create folder failed</source>
<translation>Echec de la création du dossier</translation>
</message>
<message>
<location filename="../src/filebrowser/file-browser-dialog.cpp" line="740"/>
<source>Select a file to update %1</source>
<translation>Séeéctionner un fichier à mettre à jour %1</translation>
</message>
<message>
<location filename="../src/filebrowser/file-browser-dialog.cpp" line="748"/>
<source>Rename failed</source>
<translation>Échec du renommage</translation>
</message>
<message>
<location filename="../src/filebrowser/file-browser-dialog.cpp" line="763"/>
<source>Remove failed</source>
<translation>Échec de l'effacement</translation>
</message>
<message>
<location filename="../src/filebrowser/file-browser-dialog.cpp" line="773"/>
<source>Share failed</source>
<translation>Échec du partage</translation>
</message>
</context>
<context>
<name>FileBrowserProgressDialog</name>
<message>
<location filename="../src/filebrowser/progress-dialog.cpp" line="28"/>
<source>Cancel</source>
<translation>Annuler</translation>
</message>
<message>
<location filename="../src/filebrowser/progress-dialog.cpp" line="51"/>
<source>Upload</source>
<translation>Envoyer</translation>
</message>
<message>
<location filename="../src/filebrowser/progress-dialog.cpp" line="52"/>
<source>Uploading %1</source>
<translation>Envoi de %1</translation>
</message>
<message>
<location filename="../src/filebrowser/progress-dialog.cpp" line="54"/>
<source>Download</source>
<translation>Télécharger</translation>
</message>
<message>
<location filename="../src/filebrowser/progress-dialog.cpp" line="55"/>
<source>Downloading %1</source>
<translation>Téléchargement de %1</translation>
</message>
<message>
<location filename="../src/filebrowser/progress-dialog.cpp" line="79"/>
<source>%1 of %2</source>
<translation>%1 sur %2</translation>
</message>
</context>
<context>
<name>FileNetworkTask</name>
<message>
<location filename="../src/filebrowser/tasks.cpp" line="124"/>
<source>Operation canceled</source>
<translation>Opération annulée</translation>
</message>
<message>
<location filename="../src/filebrowser/tasks.cpp" line="168"/>
<source>pending</source>
<translation>en cours</translation>
</message>
</context>
<context>
<name>FileServerTask</name>
<message>
<location filename="../src/filebrowser/tasks.cpp" line="665"/>
<source>Internal Server Error</source>
<translation>Erreur interne du serveur</translation>
</message>
</context>
<context>
<name>FileTableModel</name>
<message>
<location filename="../src/filebrowser/file-table.cpp" line="509"/>
<source>Folder</source>
<translation>Dossier</translation>
</message>
<message>
<location filename="../src/filebrowser/file-table.cpp" line="509"/>
<source>Document</source>
<translation>Document</translation>
</message>
<message>
<location filename="../src/filebrowser/file-table.cpp" line="539"/>
<source>Name</source>
<translation>Nom</translation>
</message>
<message>
<location filename="../src/filebrowser/file-table.cpp" line="541"/>
<source>Size</source>
<translation>Taille</translation>
</message>
<message>
<location filename="../src/filebrowser/file-table.cpp" line="543"/>
<source>Last Modified</source>
<translation>Dernière modification</translation>
</message>
<message>
<location filename="../src/filebrowser/file-table.cpp" line="545"/>
<source>Kind</source>
<translation>Type</translation>
</message>
</context>
<context>
<name>FileTableView</name>
<message>
<location filename="../src/filebrowser/file-table.cpp" line="194"/>
<location filename="../src/filebrowser/file-table.cpp" line="281"/>
<source>&Open</source>
<translation>&Ouvrir</translation>
</message>
<message>
<location filename="../src/filebrowser/file-table.cpp" line="199"/>
<source>&Rename</source>
<translation>&Renommer</translation>
</message>
<message>
<location filename="../src/filebrowser/file-table.cpp" line="203"/>
<source>&Delete</source>
<translation>&Effacer</translation>
</message>
<message>
<location filename="../src/filebrowser/file-table.cpp" line="207"/>
<source>&Generate Share Link</source>
<translation>&Générer un lien de partage</translation>
</message>
<message>
<location filename="../src/filebrowser/file-table.cpp" line="211"/>
<source>&Update</source>
<translation>&Mise à jour</translation>
</message>
<message>
<location filename="../src/filebrowser/file-table.cpp" line="214"/>
<source>&Cancel Download</source>
<translation>&Annuler le téléchargement</translation>
</message>
<message>
<location filename="../src/filebrowser/file-table.cpp" line="257"/>
<source>&Download</source>
<translation>&Télécharger</translation>
</message>
<message>
<location filename="../src/filebrowser/file-table.cpp" line="285"/>
<source>D&ownload</source>
<translation>T&éléchargement</translation>
</message>
</context>
<context>
<name>GetFileTask</name>
<message>
<location filename="../src/filebrowser/tasks.cpp" line="374"/>
<location filename="../src/filebrowser/tasks.cpp" line="415"/>
<source>Failed to create folders</source>
<translation>Échec de création des dossiers</translation>
</message>
<message>
<location filename="../src/filebrowser/tasks.cpp" line="383"/>
<source>Failed to create temporary files</source>
<translation>Échec de création des fichiers temporaires</translation>
</message>
<message>
<location filename="../src/filebrowser/tasks.cpp" line="430"/>
<source>Failed to write file to disk</source>
<translation>Échec d’écriture du fichier sur le disque</translation>
</message>
<message>
<location filename="../src/filebrowser/tasks.cpp" line="436"/>
<source>Failed to remove the older version of the downloaded file</source>
<translation>Échec de la suppression d'une ancienne version du fichier téléchargé</translation>
</message>
<message>
<location filename="../src/filebrowser/tasks.cpp" line="442"/>
<source>Failed to move file</source>
<translation>Échec du déplacement de fichier</translation>
</message>
</context>
<context>
<name>InitSeafileDialog</name>
<message>
<location filename="../src/ui/init-seafile-dialog.cpp" line="66"/>
<source>%1 Initialization</source>
<translation>Initalisation de %1</translation>
</message>
<message>
<location filename="../src/ui/init-seafile-dialog.cpp" line="96"/>
<location filename="../src/ui/init-seafile-dialog.cpp" line="111"/>
<source>Please choose a directory</source>
<translation>Veuillez choisir un répertoire</translation>
</message>
<message>
<location filename="../src/ui/init-seafile-dialog.cpp" line="139"/>
<location filename="../src/ui/init-seafile-dialog.cpp" line="150"/>
<source>Initialization is not finished. Really quit?</source>
<translation>L'initialisation n'est pas terminée. Voulez vous réellement quitter?</translation>
</message>
<message>
<location filename="../src/ui/init-seafile-dialog.cpp" line="119"/>
<source>The folder %1 does not exist</source>
<translation>Le dossier %1 n'existe pas</translation>
</message>
<message>
<location filename="../ui_init-seafile-dialog.h" line="190"/>
<source>Dialog</source>
<translation>Dialogue</translation>
</message>
<message>
<location filename="../ui_init-seafile-dialog.h" line="191"/>
<source>Choose Seafile folder</source>
<translation>Choisissez le dossier Seafile</translation>
</message>
<message>
<location filename="../ui_init-seafile-dialog.h" line="192"/>
<source>logo</source>
<translation>logo</translation>
</message>
<message>
<location filename="../ui_init-seafile-dialog.h" line="193"/>
<source>Please choose a folder. We will create a Seafile subfolder in it. When you download a library, it will be saved there by default.</source>
<translation>Choisissez un dossier. Un sous dossier Seafifle y sera créé. Quand vous téléchargez une bibliothèque, elle y sera enregistrée par défaut.</translation>
</message>
<message>
<location filename="../ui_init-seafile-dialog.h" line="194"/>
<source>Choose...</source>
<translation>Choisir...</translation>
</message>
<message>
<location filename="../ui_init-seafile-dialog.h" line="195"/>
<source>Next</source>
<translation>Suivant</translation>
</message>
<message>
<location filename="../ui_init-seafile-dialog.h" line="196"/>
<source>Cancel</source>
<translation>Annuler</translation>
</message>
</context>
<context>
<name>InitVirtualDriveDialog</name>
<message>
<location filename="../src/ui/init-vdrive-dialog.cpp" line="68"/>
<source>Checking your default library...</source>
<translation>Vérification de votre bibliothèque par défaut...</translation>
</message>
<message>
<location filename="../src/ui/init-vdrive-dialog.cpp" line="82"/>
<source>Creating the default library...</source>
<translation>Création de la bibliothèque par défaut</translation>
</message>
<message>
<location filename="../src/ui/init-vdrive-dialog.cpp" line="133"/>
<location filename="../src/ui/init-vdrive-dialog.cpp" line="149"/>
<source>Failed to create default library:
The server version must be 2.1 or higher to support this.</source>
<translation>Impossible de créer la bibliothèque par défaut:
La version de votre serveur doit être 2.1 ou plus.</translation>
</message>
<message>
<location filename="../src/ui/init-vdrive-dialog.cpp" line="136"/>
<source>Failed to get default library:
%1</source>
<translation>Impossible de récupérer la bibliothèque par défaut:
%1</translation>
</message>
<message>
<location filename="../src/ui/init-vdrive-dialog.cpp" line="152"/>
<source>Failed to create default library:
%1</source>
<translation>Impossible de créer la bibliothèque par défaut:
%1</translation>
</message>
<message>
<location filename="../src/ui/init-vdrive-dialog.cpp" line="179"/>
<source>Downloading default library...</source>
<translation>Téléchargement de la bibliothèque par défaut...</translation>
</message>
<message>
<location filename="../src/ui/init-vdrive-dialog.cpp" line="188"/>
<source>Failed to download default library:
%1</source>
<translation>Impossible de télécharger la bibliothèque par défaut:
%1</translation>
</message>
<message>
<location filename="../src/ui/init-vdrive-dialog.cpp" line="199"/>
<source>The default library has been downloaded.
You can click the "Open" button to view it.</source>
<translation>La bibliothèque par défaut a été téléchargée.
Vous pouvez cliquer sur le boutton "Ouvrir" pour la voir.</translation>
</message>
<message>
<location filename="../src/ui/init-vdrive-dialog.cpp" line="250"/>
<source>Error when downloading the default library: %1</source>
<translation>Erreur lors du téléchargement de la bibliothèque par défaut: %1</translation>
</message>
<message>
<location filename="../src/ui/init-vdrive-dialog.cpp" line="264"/>
<source>Creating the virtual disk...</source>
<translation>Création du disque virtuel...</translation>
</message>
<message>
<location filename="../src/ui/init-vdrive-dialog.cpp" line="173"/>
<source>Failed to download default library:
%1</source>
<translation>Impossible de télécharger la bibliothèque par défaut:
%1</translation>
</message>
<message>
<location filename="../ui_init-vdrive-dialog.h" line="185"/>
<source>Dialog</source>
<translation>Dialogue</translation>
</message>
<message>
<location filename="../ui_init-vdrive-dialog.h" line="188"/>
<source>Seafile organizes files by libraries.
Do you like to download your default library and create a virtual disk?</source>
<translation>Seafile organise les fichiers par bibliothèques.
Voulez vous télécharger la bibliothèque par défaut et créer un disque virtuel?</translation>
</message>
<message>
<location filename="../ui_init-vdrive-dialog.h" line="191"/>
<source>Skip</source>
<translation>Passer</translation>
</message>
<message>
<location filename="../ui_init-vdrive-dialog.h" line="192"/>
<source>Run in Background</source>
<translation>Lancer en arrière plan</translation>
</message>
<message>
<location filename="../ui_init-vdrive-dialog.h" line="193"/>
<source>Open</source>
<translation>Ouvrir</translation>
</message>
<message>
<location filename="../ui_init-vdrive-dialog.h" line="194"/>
<source>Finish</source>
<translation>Terminer</translation>
</message>
<message>
<location filename="../src/ui/init-vdrive-dialog.cpp" line="34"/>
<location filename="../ui_init-vdrive-dialog.h" line="186"/>
<source>Download Default Library</source>
<translation>Télécharger la bibliothèque par défaut</translation>
</message>
<message>
<location filename="../ui_init-vdrive-dialog.h" line="190"/>
<source>Yes</source>
<translation>Oui</translation>
</message>
<message>
<location filename="../ui_init-vdrive-dialog.h" line="187"/>
<source>logo</source>
<translation>logo</translation>
</message>
</context>
<context>
<name>LoginDialog</name>
<message>
<location filename="../src/ui/login-dialog.cpp" line="22"/>
<location filename="../ui_login-dialog.h" line="257"/>
<source>Add an account</source>
<translation>Ajouter un compte</translation>
</message>
<message>
<location filename="../src/ui/login-dialog.cpp" line="50"/>
<source>Logging in...</source>
<translation>Connexion...</translation>
</message>
<message>
<location filename="../src/ui/login-dialog.cpp" line="88"/>
<source>Network Error:
%1</source>
<translation>Erreur réseau : %1</translation>
</message>
<message>
<location filename="../src/ui/login-dialog.cpp" line="100"/>
<source><b>Warning:</b> The ssl certificate of this server is not trusted, proceed anyway?</source>
<translation><b>Attention :</b> Le certificat de sécurité de ce serveur n'est pas approuvé, continuer quand même ?</translation>
</message>
<message>
<location filename="../src/ui/login-dialog.cpp" line="114"/>
<source>Please enter the server address</source>
<translation>Veuillez entrer l'adresse du serveur</translation>
</message>
<message>
<location filename="../src/ui/login-dialog.cpp" line="118"/>
<location filename="../src/ui/login-dialog.cpp" line="124"/>
<source>%1 is not a valid server address</source>
<translation>%1 n'est pas une adresse de serveur valide</translation>
</message>
<message>
<location filename="../src/ui/login-dialog.cpp" line="131"/>
<source>Please enter the username</source>
<translation>Veuillez entrer le nom d'utilisateur</translation>
</message>
<message>
<location filename="../src/ui/login-dialog.cpp" line="142"/>
<source>Please enter the computer name</source>
<translation>Veuillez entrer le nom de l'ordinateur</translation>
</message>
<message>
<location filename="../src/ui/login-dialog.cpp" line="159"/>
<source>Failed to save current account</source>
<translation>Impossible d'enregistrer le compte actuel</translation>
</message>
<message>
<location filename="../src/ui/login-dialog.cpp" line="136"/>
<source>Please enter the password</source>
<translation>Veuillez entrer le mot de passe</translation>
</message>
<message>
<location filename="../src/ui/login-dialog.cpp" line="186"/>
<source>Incorrect email or password</source>
<translation>Email ou mot de passe incorrect</translation>
</message>
<message>
<location filename="../src/ui/login-dialog.cpp" line="188"/>
<source>Logging in too frequently, please wait a minute</source>
<translation>Connexions trop fréquentes, veuillez attendre une minute</translation>
</message>
<message>
<location filename="../src/ui/login-dialog.cpp" line="190"/>
<source>Internal Server Error</source>
<translation>Erreur interne du serveur</translation>
</message>
<message>
<location filename="../src/ui/login-dialog.cpp" line="194"/>
<source>Failed to login: %1</source>
<translation>Impossible de se connecter:%1</translation>
</message>
<message>
<location filename="../src/ui/login-dialog.cpp" line="196"/>
<source>Failed to login</source>
<translation>Impossible de se connecter</translation>
</message>
<message>
<location filename="../ui_login-dialog.h" line="256"/>
<source>Dialog</source>
<translation>Dialogue</translation>
</message>
<message>
<location filename="../ui_login-dialog.h" line="258"/>
<source>logo</source>
<translation>logo</translation>
</message>
<message>
<location filename="../ui_login-dialog.h" line="259"/>
<source>Server:</source>
<translation>Serveur:</translation>
</message>
<message>
<location filename="../ui_login-dialog.h" line="260"/>
<source><html><head/><body><p>For example: https://seacloud.cc</p></body></html></source>
<translation><html><head/><body><p>Par exemple: https://seacloud.cc</p></body></html></translation>
</message>
<message>
<location filename="../ui_login-dialog.h" line="261"/>
<source>or http://192.168.1.24:8000</source>
<translation>ou http://192.168.1.24:8000</translation>
</message>
<message>
<location filename="../ui_login-dialog.h" line="262"/>
<source>Email:</source>
<translation>Email :</translation>
</message>
<message>
<location filename="../ui_login-dialog.h" line="263"/>
<source>Password:</source>
<translation>Mot de passe :</translation>
</message>
<message>
<location filename="../ui_login-dialog.h" line="266"/>
<source>status text</source>
<translation>texte d'état</translation>
</message>
<message>
<location filename="../ui_login-dialog.h" line="264"/>
<source>Computer Name:</source>
<translation>Nom de l'ordinateur :</translation>
</message>
<message>
<location filename="../ui_login-dialog.h" line="265"/>
<source>e.g. Jim's laptop</source>
<translation>par ex : portable de Julien</translation>
</message>
<message>
<location filename="../ui_login-dialog.h" line="267"/>
<source>Login</source>
<translation>Connexion</translation>
</message>
<message>
<location filename="../ui_login-dialog.h" line="268"/>
<source>Cancel</source>
<translation>Annuler</translation>
</message>
</context>
<context>
<name>MainWindow</name>
<message>
<location filename="../src/ui/main-window.cpp" line="151"/>
<source>Refresh</source>
<translation>Rafraîchir</translation>
</message>
</context>
<context>
<name>MessageListener</name>
<message>
<location filename="../src/message-listener.cpp" line="85"/>
<source>failed to load ccnet config dir </source>
<translation>Impossible de charger la configuration de ccnet</translation>
</message>
<message>
<location filename="../src/message-listener.cpp" line="163"/>
<source>"%1" is unsynced.
Reason: Deleted on server</source>
<translation>"%1" est désynchronisé.
Raison : Supprimé sur le serveur</translation>
</message>
<message>
<location filename="../src/message-listener.cpp" line="173"/>
<source>"%1" is synchronized</source>
<translation>"%1" est synchronisé</translation>
</message>
<message>
<location filename="../src/message-listener.cpp" line="185"/>
<source>"%1" failed to sync.
Access denied to service</source>
<translation>"%1" impossible à synchroniser.
Accès refusé au service</translation>
</message>
<message>
<location filename="../src/message-listener.cpp" line="196"/>
<source>"%1" failed to sync.
The library owner's storage space is used up.</source>
<translation>"%1" impossible de synchroniser.
L'espace de stockage du propriétaire de la bibliothèque est plein.</translation>
</message>
</context>
<context>
<name>PostFileTask</name>
<message>
<location filename="../src/filebrowser/tasks.cpp" line="486"/>
<location filename="../src/filebrowser/tasks.cpp" line="491"/>
<source>File does not exist</source>
<translation>Le fichier n'existe pas</translation>
</message>
</context>
<context>
<name>QObject</name>
<message>
<location filename="../src/message-listener.cpp" line="52"/>
<source>Uploading</source>
<translation>Envoi</translation>
</message>
<message>
<location filename="../src/message-listener.cpp" line="52"/>
<source>Downloading</source>
<translation>Téléchargement</translation>
</message>
<message>
<location filename="../src/message-listener.cpp" line="53"/>
<source>Speed</source>
<translation>Vitesse</translation>
</message>
<message>
<location filename="../src/rpc/local-repo.cpp" line="68"/>
<source>synchronized</source>
<translation>Synchronisé</translation>
</message>
<message>
<location filename="../src/rpc/local-repo.cpp" line="72"/>
<source>indexing files</source>
<translation>indexation des fichiers</translation>
</message>
<message>
<location filename="../src/rpc/local-repo.cpp" line="76"/>
<source>sync initializing</source>
<translation>initialisation de la synchronisation</translation>
</message>
<message>
<location filename="../src/rpc/local-repo.cpp" line="80"/>
<source>downloading</source>
<translation>Téléchargement</translation>
</message>
<message>
<location filename="../src/rpc/local-repo.cpp" line="84"/>
<source>uploading</source>
<translation>Envoi</translation>
</message>
<message>
<location filename="../src/rpc/local-repo.cpp" line="88"/>
<source>sync merging</source>
<translation>fusion de la synchronisation</translation>
</message>
<message>
<location filename="../src/rpc/local-repo.cpp" line="92"/>
<source>waiting for sync</source>
<translation>en attente de synchronisation</translation>
</message>
<message>
<location filename="../src/rpc/local-repo.cpp" line="96"/>
<location filename="../src/rpc/local-repo.cpp" line="124"/>
<source>server not connected</source>
<translation>serveur non connecté</translation>
</message>
<message>
<location filename="../src/rpc/local-repo.cpp" line="100"/>
<source>server authenticating</source>
<translation>authentification du serveur</translation>
</message>
<message>
<location filename="../src/rpc/local-repo.cpp" line="104"/>
<location filename="../src/rpc/local-repo.cpp" line="114"/>
<source>auto sync is turned off</source>
<translation>La synchronisation automatique est désactivée</translation>
</message>
<message>
<location filename="../src/rpc/local-repo.cpp" line="109"/>
<source>unknown</source>
<translation>inconnu</translation>
</message>
<message>
<location filename="../src/rpc/local-repo.cpp" line="127"/>
<source>Server has been removed</source>
<translation>Le serveur a été supprimé</translation>
</message>
<message>
<location filename="../src/rpc/local-repo.cpp" line="130"/>
<source>You have not logged in to the server</source>
<translation>Vous n'êtes pas connecté sur le serveur</translation>
</message>
<message>
<location filename="../src/rpc/local-repo.cpp" line="133"/>
<source>You do not have permission to access this library</source>
<translation>Vous n'avez pas l'autorisation d'accéder à cette bibliothèque</translation>
</message>
<message>
<location filename="../src/rpc/local-repo.cpp" line="136"/>
<source>The storage space of the library owner has been used up</source>
<translation>L'espace de stockage du propriétaire de cette bibliothèque est plein</translation>
</message>
<message>
<location filename="../src/rpc/local-repo.cpp" line="139"/>
<source>Remote service is not available</source>
<translation>Le service distant n'est pas disponible</translation>
</message>
<message>
<location filename="../src/rpc/local-repo.cpp" line="142"/>
<location filename="../src/rpc/local-repo.cpp" line="184"/>
<source>Access denied to service</source>
<translation>Accès refusé au service.</translation>
</message>
<message>
<location filename="../src/rpc/local-repo.cpp" line="145"/>
<source>Internal data corrupted</source>
<translation>Donnée interne corrompue</translation>
</message>
<message>
<location filename="../src/rpc/local-repo.cpp" line="148"/>
<source>Failed to start upload</source>
<translation>Erreur au démarrage de l'envoi</translation>
</message>
<message>
<location filename="../src/rpc/local-repo.cpp" line="151"/>
<source>Error occured in upload</source>
<translation>Erreur pendant l'envoi</translation>
</message>
<message>
<location filename="../src/rpc/local-repo.cpp" line="154"/>
<source>Failed to start download</source>
<translation>Echec au démarrage du téléchargement</translation>
</message>
<message>
<location filename="../src/rpc/local-repo.cpp" line="157"/>
<source>Error occured in download</source>
<translation>Erreur pendant le téléchargement</translation>
</message>
<message>
<location filename="../src/rpc/local-repo.cpp" line="163"/>
<source>Library is damaged on server</source>
<translation>La librairie est endommagée sur le serveur</translation>
</message>
<message>
<location filename="../src/rpc/local-repo.cpp" line="166"/>
<source>Conflict in merge</source>
<translation>Conflit pendant la fusion</translation>
</message>
<message>
<location filename="../src/rpc/local-repo.cpp" line="169"/>
<source>Server version is too old</source>
<translation>La version du serveur est trop ancienne</translation>
</message>
<message>
<location filename="../src/rpc/local-repo.cpp" line="175"/>
<source>Unknown error</source>
<translation>Erreur inconnue</translation>
</message>
<message>
<location filename="../src/rpc/local-repo.cpp" line="178"/>
<source>The storage quota has been used up</source>
<translation>Le quota de stockage est atteint</translation>
</message>
<message>
<location filename="../src/rpc/local-repo.cpp" line="181"/>
<source>Internal server error</source>
<translation>Erreur interne du serveur</translation>
</message>
<message>
<location filename="../src/rpc/local-repo.cpp" line="187"/>
<source>Your %1 client is too old</source>
<translation>Votre client %1 est trop ancien</translation>
</message>
<message>
<location filename="../src/rpc/local-repo.cpp" line="190"/>
<location filename="../src/rpc/local-repo.cpp" line="193"/>
<location filename="../src/rpc/local-repo.cpp" line="196"/>
<location filename="../src/rpc/local-repo.cpp" line="199"/>
<location filename="../src/rpc/local-repo.cpp" line="202"/>
<location filename="../src/rpc/local-repo.cpp" line="204"/>
<location filename="../src/rpc/local-repo.cpp" line="206"/>
<location filename="../src/rpc/local-repo.cpp" line="208"/>
<location filename="../src/rpc/local-repo.cpp" line="210"/>
<location filename="../src/rpc/local-repo.cpp" line="212"/>
<location filename="../src/rpc/local-repo.cpp" line="214"/>
<location filename="../src/rpc/local-repo.cpp" line="216"/>
<location filename="../src/rpc/local-repo.cpp" line="218"/>
<location filename="../src/rpc/local-repo.cpp" line="220"/>
<location filename="../src/rpc/local-repo.cpp" line="222"/>
<location filename="../src/rpc/local-repo.cpp" line="224"/>
<location filename="../src/rpc/local-repo.cpp" line="226"/>
<location filename="../src/rpc/local-repo.cpp" line="228"/>
<location filename="../src/rpc/local-repo.cpp" line="230"/>
<location filename="../src/rpc/local-repo.cpp" line="232"/>
<location filename="../src/rpc/local-repo.cpp" line="234"/>
<location filename="../src/rpc/local-repo.cpp" line="236"/>
<source>Failed to sync this library</source>
<translation>Echec de synchronisation de cette librairie</translation>
</message>
<message>
<location filename="../src/rpc/local-repo.cpp" line="239"/>
<source>Files are locked by other application</source>
<translation>Les fichiers sont ouverts par une autre application</translation>
</message>
<message>
<location filename="../src/rpc/local-repo.cpp" line="160"/>
<source>Library is deleted on server</source>
<translation>La bibliothèque a été supprimée sur le serveur</translation>
</message>
<message>
<location filename="../src/rpc/local-repo.cpp" line="172"/>
<source>Error when accessing the local folder</source>
<translation>Impossible d'accéder au répertoire local</translation>
</message>
<message>
<location filename="../src/rpc/clone-task.cpp" line="71"/>
<source>initializing...</source>
<translation>initalisation...</translation>
</message>
<message>
<location filename="../src/rpc/clone-task.cpp" line="74"/>
<source>connecting server...</source>
<translation>connexion au serveur...</translation>
</message>
<message>
<location filename="../src/rpc/clone-task.cpp" line="77"/>
<source>indexing files...</source>
<translation>indexation des fichiers...</translation>
</message>
<message>
<location filename="../src/rpc/clone-task.cpp" line="80"/>
<source>Downloading...</source>
<translation>Téléchargement...</translation>
</message>
<message>
<location filename="../src/rpc/clone-task.cpp" line="86"/>
<source>Creating folder...</source>
<translation>Création du dossier...</translation>
</message>
<message>
<location filename="../src/rpc/clone-task.cpp" line="92"/>
<source>Merge file changes...</source>
<translation>Fusion des changements des fichiers...</translation>
</message>
<message>
<location filename="../src/rpc/clone-task.cpp" line="95"/>
<source>Done</source>
<translation>Terminé</translation>
</message>
<message>
<location filename="../src/rpc/clone-task.cpp" line="98"/>
<source>Canceling</source>
<translation>Annulation</translation>
</message>
<message>
<location filename="../src/rpc/clone-task.cpp" line="101"/>
<source>Canceled</source>
<translation>Annulé</translation>
</message>
<message>
<location filename="../src/rpc/clone-task.cpp" line="105"/>
<source>Failed to index local files.</source>
<translation>Impossible d'indexer les fichiers locaux.</translation>
</message>
<message>
<location filename="../src/rpc/clone-task.cpp" line="108"/>
<source>Failed to create local files.</source>
<translation>Impossible de créer les fichiers locaux.</translation>
</message>
<message>
<location filename="../src/rpc/clone-task.cpp" line="111"/>
<source>Failed to merge local file changes.</source>
<translation>Impossible de fusionner les changements des fichiers locaux.</translation>
</message>
<message>
<location filename="../src/rpc/clone-task.cpp" line="114"/>
<source>Incorrect password. Please download again.</source>
<translation>Mot de passe incorrect. Veuillez télécharger à nouveau.</translation>
</message>
<message>
<location filename="../src/rpc/clone-task.cpp" line="116"/>
<source>Internal error.</source>
<translation>Erreur interne.</translation>
</message>
<message>
<location filename="../src/main.cpp" line="128"/>
<source>%1 is already running</source>
<translation>%1 fonctionne déjà</translation>
</message>
<message>
<location filename="../src/api/api-error.cpp" line="55"/>
<source>SSL Error</source>
<translation>Erreur SSL</translation>
</message>
<message>
<location filename="../src/api/api-error.cpp" line="57"/>
<source>Network Error: %1</source>
<translation>Erreur Réseau: %1</translation>
</message>
<message>
<location filename="../src/api/api-error.cpp" line="59"/>
<source>Server Error</source>
<translation>Erreur du Serveur</translation>
</message>
<message>
<location filename="../src/certs-mgr.cpp" line="77"/>
<source>failed to open certs database</source>
<translation>Echec d'ouverture de la base des certificats</translation>
</message>
<message>
<location filename="../src/open-local-helper.cpp" line="161"/>
<source>The library "%1" has not been synced yet</source>
<translation>La bibliothèque %1" n'a pas encore été synchronisée.</translation>
</message>
<message>
<location filename="../src/open-local-helper.cpp" line="172"/>
<location filename="../src/repo-service-helper.cpp" line="20"/>
<source>%1 couldn't find an application to open file %2</source>
<translation>%1 ne trouve pas d'application pour ouvir le fichier %2</translation>
</message>
<message>
<location filename="../src/api/event.cpp" line="50"/>
<source>Created library "%1"</source>
<translation>Bibliothèque "%1" créée</translation>
</message>
<message>
<location filename="../src/api/event.cpp" line="52"/>
<source>Deleted library "%1"</source>
<translation>Bibliothèque "%1" supprimée</translation>
</message>
<message>
<location filename="../src/filebrowser/data-cache.cpp" line="87"/>
<source>failed to open file cache database</source>
<translation>Échec de l'ouverture du fichier cache de la base de données</translation>
</message>
<message>
<location filename="../src/filebrowser/file-browser-dialog.cpp" line="664"/>
<source>Rename %1 to</source>
<translation>Renommer %1 en </translation>
</message>
<message>
<location filename="../src/repo-service-helper.cpp" line="110"/>
<source>Unable to download item "%1"</source>
<translation>Impossible de télécharger l'objet "%1"</translation>
</message>
<message>
<location filename="../src/ui/repo-tree-view.cpp" line="854"/>
<source>copy failed</source>
<translation>Echec de la copie</translation>
</message>
</context>
<context>
<name>RepoDetailDialog</name>
<message>
<location filename="../src/ui/repo-detail-dialog.cpp" line="29"/>
<source>Library "%1"</source>
<translation>Bibliothèque"%1"</translation>
</message>
<message>
<location filename="../src/ui/repo-detail-dialog.cpp" line="65"/>
<location filename="../src/ui/repo-detail-dialog.cpp" line="127"/>
<source>This library is not downloaded yet</source>
<translation>Cette bibliothèque n'a pas encore été téléchargée</translation>
</message>
<message>
<location filename="../src/ui/repo-detail-dialog.cpp" line="92"/>
<source>Error: </source>
<translation>Erreur : </translation>
</message>
<message>
<location filename="../ui_repo-detail-dialog.h" line="215"/>
<source>Dialog</source>
<translation>Dialogue</translation>
</message>
<message>
<location filename="../ui_repo-detail-dialog.h" line="216"/>
<source>RepoIcon</source>
<translation>IconeRepo</translation>
</message>
<message>
<location filename="../ui_repo-detail-dialog.h" line="217"/>
<source>RepoName</source>
<translation>NomRepo</translation>
</message>
<message>
<location filename="../ui_repo-detail-dialog.h" line="218"/>
<source>Description:</source>
<translation>Description :</translation>
</message>
<message>
<location filename="../ui_repo-detail-dialog.h" line="219"/>
<location filename="../ui_repo-detail-dialog.h" line="221"/>
<location filename="../ui_repo-detail-dialog.h" line="225"/>
<location filename="../ui_repo-detail-dialog.h" line="227"/>
<source>TextLabel</source>
<translation>LabelTexte</translation>
</message>
<message>
<location filename="../ui_repo-detail-dialog.h" line="220"/>
<source>Owner:</source>
<translation>Propriétaire :</translation>
</message>
<message>
<location filename="../ui_repo-detail-dialog.h" line="222"/>
<source>Last Modified:</source>
<translation>Dernière modification:</translation>
</message>
<message>
<location filename="../ui_repo-detail-dialog.h" line="223"/>
<source>mtime</source>
<translation>mtime</translation>
</message>
<message>
<location filename="../ui_repo-detail-dialog.h" line="224"/>
<source>Size:</source>
<translation>Taille :</translation>
</message>
<message>
<location filename="../ui_repo-detail-dialog.h" line="226"/>
<source>Local Path:</source>
<translation>Répertoire local :</translation>
</message>
<message>
<location filename="../ui_repo-detail-dialog.h" line="228"/>
<source>Status:</source>
<translation>État :</translation>
</message>
<message>
<location filename="../ui_repo-detail-dialog.h" line="229"/>
<source>RepoStatus</source>
<translation>StatutRepo</translation>
</message>
<message>
<location filename="../ui_repo-detail-dialog.h" line="230"/>
<source>Name:</source>
<translation>Nom :</translation>
</message>
<message>
<location filename="../ui_repo-detail-dialog.h" line="231"/>
<source>Close</source>
<translation>Fermer</translation>
</message>
</context>
<context>
<name>RepoItemDelegate</name>
<message>
<location filename="../src/ui/repo-item-delegate.cpp" line="371"/>
<source>This library has not been downloaded</source>
<translation>Cette bibliothèque n'a pas été téléchargée</translation>
</message>
</context>
<context>
<name>RepoTreeModel</name>
<message>
<location filename="../src/ui/repo-tree-model.cpp" line="69"/>
<source>Recently Updated</source>
<translation>Mis à jour récemment</translation>
</message>
<message>
<location filename="../src/ui/repo-tree-model.cpp" line="70"/>
<source>My Libraries</source>
<translation>Mes bibliothèques</translation>
</message>
<message>
<location filename="../src/ui/repo-tree-model.cpp" line="71"/>
<source>Sub Libraries</source>
<translation>Sous bibliothèques</translation>
</message>
<message>
<location filename="../src/ui/repo-tree-model.cpp" line="72"/>
<source>Private Shares</source>
<translation>Partages privés</translation>
</message>
<message>
<location filename="../src/ui/repo-tree-model.cpp" line="242"/>
<source>Organization</source>
<translation>Organisation</translation>
</message>
</context>
<context>
<name>RepoTreeView</name>
<message>
<location filename="../src/ui/repo-tree-view.cpp" line="264"/>
<location filename="../src/ui/repo-tree-view.cpp" line="265"/>
<source>Disable auto sync</source>
<translation>Désactiver la synchronisation automatique</translation>
</message>
<message>
<location filename="../src/ui/repo-tree-view.cpp" line="268"/>
<location filename="../src/ui/repo-tree-view.cpp" line="269"/>
<location filename="../src/ui/repo-tree-view.cpp" line="382"/>
<location filename="../src/ui/repo-tree-view.cpp" line="383"/>
<source>Enable auto sync</source>
<translation>Activer la synchronisation automatique</translation>
</message>
<message>
<location filename="../src/ui/repo-tree-view.cpp" line="329"/>
<source>Show details of this library</source>
<translation>Afficher les détails de cette bibliothèque</translation>
</message>
<message>
<location filename="../src/ui/repo-tree-view.cpp" line="334"/>
<location filename="../src/ui/repo-tree-view.cpp" line="341"/>
<source>&Sync this library</source>
<translation>&Synchroniser cette bibliothèque</translation>
</message>
<message>
<location filename="../src/ui/repo-tree-view.cpp" line="336"/>
<location filename="../src/ui/repo-tree-view.cpp" line="343"/>
<source>Sync this library</source>
<translation>Synchroniser cette bibliothèque</translation>
</message>
<message>
<location filename="../src/ui/repo-tree-view.cpp" line="327"/>
<source>Show &Details</source>
<translation>Afficher les &détails</translation>
</message>
<message>
<location filename="../src/ui/repo-tree-view.cpp" line="148"/>
<source>Recently Updated</source>
<translation>Récemment envoyés</translation>
</message>
<message>
<location filename="../src/ui/repo-tree-view.cpp" line="348"/>
<source>Sync &Now</source>
<translation>Synchroniser &maintenant</translation>
</message>
<message>
<location filename="../src/ui/repo-tree-view.cpp" line="350"/>
<source>Sync this library immediately</source>
<translation>Synchroniser cette bibliothèque immédiatement</translation>
</message>
<message>
<location filename="../src/ui/repo-tree-view.cpp" line="355"/>
<source>&Cancel download</source>
<translation>&Annuler le téléchargement</translation>
</message>
<message>
<location filename="../src/ui/repo-tree-view.cpp" line="357"/>
<source>Cancel download of this library</source>
<translation>Annuler le téléchargement de cette bibliothèque</translation>
</message>
<message>
<location filename="../src/ui/repo-tree-view.cpp" line="362"/>
<location filename="../src/ui/repo-tree-view.cpp" line="369"/>
<source>&Open folder</source>
<translation>&Ouvrir le dossier local</translation>
</message>
<message>
<location filename="../src/ui/repo-tree-view.cpp" line="364"/>
<location filename="../src/ui/repo-tree-view.cpp" line="371"/>
<source>open local folder</source>
<translation>ouvrir le dossier local</translation>
</message>
<message>
<location filename="../src/ui/repo-tree-view.cpp" line="376"/>
<source>&Unsync</source>
<translation>&Désynchroniser</translation>
</message>
<message>
<location filename="../src/ui/repo-tree-view.cpp" line="377"/>
<source>unsync this library</source>
<translation>désynchroniser cettte bibliothèque</translation>
</message>
<message>
<location filename="../src/ui/repo-tree-view.cpp" line="388"/>
<source>&View on cloud</source>
<translation>Voir dans le &cloud</translation>
</message>
<message>
<location filename="../src/ui/repo-tree-view.cpp" line="390"/>
<source>view this library on seahub</source>
<translation>voir cette bibliothèque sur seahub</translation>
</message>
<message>
<location filename="../src/ui/repo-tree-view.cpp" line="396"/>
<source>&Resync this library</source>
<translation>&Resynchroniser cette bibliothèque</translation>
</message>
<message>
<location filename="../src/ui/repo-tree-view.cpp" line="398"/>
<source>unsync and resync this library</source>
<translation>désynchroniser et resynchroniser cette bibliothèque</translation>
</message>
<message>
<location filename="../src/ui/repo-tree-view.cpp" line="439"/>
<source>Are you sure to unsync library "%1"?</source>
<translation>Êtes-vous certain de vouloir désynchroniser la bibliothèque "%1" ?</translation>
</message>
<message>
<location filename="../src/ui/repo-tree-view.cpp" line="451"/>
<location filename="../src/ui/repo-tree-view.cpp" line="692"/>
<source>Failed to unsync library "%1"</source>
<translation>Impossible de désynchroniser la bibliothèque "%1"</translation>
</message>
<message>
<location filename="../src/ui/repo-tree-view.cpp" line="628"/>
<source>Failed to cancel this task:
%1</source>
<translation>Impossible d'annuler cette tâche:
%1</translation>
</message>
<message>
<location filename="../src/ui/repo-tree-view.cpp" line="632"/>
<source>The download has been canceled</source>
<translation>Le téléchargement a été annulé</translation>
</message>
<message>
<location filename="../src/ui/repo-tree-view.cpp" line="681"/>
<source>Are you sure to unsync and resync library "%1"?</source>
<translation>Voulez-vous désynchroniser et resynchroniser la bibliothèque "%1"</translation>
</message>
<message>
<location filename="../src/ui/repo-tree-view.cpp" line="718"/>
<source>Failed to add download task:
%1</source>
<translation>Échec de l'ajout du téléchargement:
%1</translation>
</message>
<message>
<location filename="../src/ui/repo-tree-view.cpp" line="752"/>
<source>Are you sure to overwrite file "%1"</source>
<translation>Voulez-vous remplacer le fichier "%1"</translation>
</message>
<message>
<location filename="../src/ui/repo-tree-view.cpp" line="755"/>
<source>Unable to delete file "%1"</source>
<translation>Impossible d'effacer le fichier "%1"</translation>
</message>
<message>
<location filename="../src/ui/repo-tree-view.cpp" line="847"/>
<source>Failed to upload file: %1</source>
<translation>Echec de l'envoi du fichier: %1</translation>
</message>
</context>
<context>
<name>ReposTab</name>
<message>
<location filename="../src/ui/repos-tab.cpp" line="40"/>
<source>Search libraries...</source>
<translation>Recherche bibliothèques...</translation>
</message>
<message>
<location filename="../src/ui/repos-tab.cpp" line="92"/>
<source>retry</source>
<translation>réessayer </translation>
</message>
<message>
<location filename="../src/ui/repos-tab.cpp" line="93"/>
<source>Failed to get libraries information<br/>Please %1</source>
<translation>Impossible de récupérer les informations des bibliothèques<br/>Veuillez %1</translation>
</message>
</context>
<context>
<name>SeafileApiClient</name>
<message>
<location filename="../src/api/api-client.cpp" line="132"/>
<source><b>Warning:</b> The ssl certificate of this server is not trusted, proceed anyway?</source>
<translation><b>Attention :</b> Le certificat de sécurité de ce serveur n'est pas approuvé, continuer quand même ?</translation>
</message>
</context>
<context>
<name>SeafileApplet</name>
<message>
<location filename="../src/seafile-applet.cpp" line="273"/>
<source>Failed to initialize log</source>
<translation>Impossible d'initialiser les logs</translation>
</message>
<message>
<location filename="../src/seafile-applet.cpp" line="386"/>
<source>A new version of %1 client (%2) is available.
Do you want to visit the download page?</source>
<translation>Une nouvelle version du client %1 (%2) est disponible.
Voulez vous visiter la page de téléchargement?</translation>
</message>
</context>
<context>
<name>SeafileRpcClient</name>
<message>
<location filename="../src/rpc/rpc-client.cpp" line="44"/>
<source>failed to load ccnet config dir %1</source>
<translation>impossible de charger le répertoire de configuration de ccnet %1 </translation>
</message>
<message>
<location filename="../src/rpc/rpc-client.cpp" line="466"/>
<location filename="../src/rpc/rpc-client.cpp" line="483"/>
<location filename="../src/rpc/rpc-client.cpp" line="547"/>
<location filename="../src/rpc/rpc-client.cpp" line="696"/>
<source>Unknown error</source>
<translation>Erreur Inconnue</translation>
</message>
<message>
<location filename="../src/rpc/rpc-client.cpp" line="662"/>
<source>The path "%1" conflicts with system path</source>
<translation>Le répertoire "%1" est en conflit avec le répertoire système</translation>
</message>
<message>
<location filename="../src/rpc/rpc-client.cpp" line="664"/>
<source>The path "%1" conflicts with an existing library</source>
<translation>Le répertoire "%1" est en conflit avec une bibliothèque existante</translation>
</message>
</context>
<context>
<name>SeafileTrayIcon</name>
<message>
<location filename="../src/ui/tray-icon.cpp" line="101"/>
<source>Disable auto sync</source>
<translation>Désactiver la synchronisation automatique</translation>
</message>
<message>
<location filename="../src/ui/tray-icon.cpp" line="104"/>
<source>Enable auto sync</source>
<translation>Activer la synchronisation automatique</translation>
</message>
<message>
<location filename="../src/ui/tray-icon.cpp" line="107"/>
<source>View unread notifications</source>
<translation>Voir les notifications non lues</translation>
</message>
<message>
<location filename="../src/ui/tray-icon.cpp" line="111"/>
<source>&Quit</source>
<translation>&Quitter</translation>
</message>
<message>
<location filename="../src/ui/tray-icon.cpp" line="114"/>
<location filename="../src/ui/tray-icon.cpp" line="166"/>
<source>Show main window</source>
<translation>Voir la fenêtre principale</translation>
</message>
<message>
<location filename="../src/ui/tray-icon.cpp" line="117"/>
<source>Settings</source>
<translation>Paramètres</translation>
</message>
<message>
<location filename="../src/ui/tray-icon.cpp" line="120"/>
<source>Open &logs folder</source>
<translation>Ouvrir le dossier &logs</translation>
</message>
<message>
<location filename="../src/ui/tray-icon.cpp" line="121"/>
<source>open seafile log directory</source>
<translation>ouvrier le répertoire des logs seafile</translation>
</message>
<message>
<location filename="../src/ui/tray-icon.cpp" line="124"/>
<source>&About</source>
<translation>À &propos</translation>
</message>
<message>
<location filename="../src/ui/tray-icon.cpp" line="125"/>
<source>Show the application's About box</source>
<translation>Voir la boite de dialogue À propos</translation>
</message>
<message>
<location filename="../src/ui/tray-icon.cpp" line="128"/>
<source>&Online help</source>
<translation>Aide en &Ligne</translation>
</message>
<message>
<location filename="../src/ui/tray-icon.cpp" line="129"/>
<source>open seafile online help</source>
<translation>ouvrir l'aide en ligne de seafile</translation>
</message>
<message>
<location filename="../src/ui/tray-icon.cpp" line="135"/>
<source>Help</source>
<translation>Aide</translation>
</message>
<message>
<location filename="../src/ui/tray-icon.cpp" line="168"/>
<source>Hide main window</source>
<translation>Cacher la fenêtre principale</translation>
</message>
<message numerus="yes">
<location filename="../src/ui/tray-icon.cpp" line="413"/>
<source>You have %n message(s)</source>
<translation><numerusform>Vous avez %n message</numerusform><numerusform>Vous avez %n message(s)</numerusform></translation>
</message>
<message>
<location filename="../src/ui/tray-icon.cpp" line="419"/>
<source>auto sync is disabled</source>
<translation>La synchronisation automatique est désactivée</translation>
</message>
<message>
<location filename="../src/ui/tray-icon.cpp" line="335"/>
<source>About %1</source>
<translation>A propos de %1</translation>
</message>
<message>
<location filename="../src/ui/tray-icon.cpp" line="336"/>
<source><h2>%1 Client %2</h2></source>
<translation><h2>%1 Client %2</h2></translation>
</message>
<message>
<location filename="../src/ui/tray-icon.cpp" line="424"/>
<source>some servers not connected</source>
<translation>des serveurs ne sont pas connectés</translation>
</message>
</context>
<context>
<name>ServerStatusDialog</name>
<message>
<location filename="../src/ui/server-status-dialog.cpp" line="13"/>
<source>Servers connection status</source>
<translation>État de connexion des serveurs</translation>
</message>
<message>
<location filename="../src/ui/server-status-dialog.cpp" line="32"/>
<source>connected</source>
<translation>connecté</translation>
</message>
<message>
<location filename="../src/ui/server-status-dialog.cpp" line="35"/>
<source>disconnected</source>
<translation>déconnecté</translation>
</message>
<message>
<location filename="../ui_server-status-dialog.h" line="70"/>
<source>Dialog</source>
<translation>Dialogue</translation>
</message>
<message>
<location filename="../ui_server-status-dialog.h" line="71"/>
<source>Close</source>
<translation>Fermer</translation>
</message>
</context>
<context>
<name>SetRepoPasswordDialog</name>
<message>
<location filename="../src/ui/set-repo-password-dialog.cpp" line="14"/>
<source>Please provide the library password</source>
<translation>Veuillez entrer le mot de passe de la bibliothèque</translation>
</message>
<message>
<location filename="../src/ui/set-repo-password-dialog.cpp" line="21"/>
<source>Provide the password for library %1</source>
<translation>Veuillez entrer le mot de passe de la bibliothèque %1</translation>
</message>
<message>
<location filename="../src/ui/set-repo-password-dialog.cpp" line="35"/>
<source>Please enter the password</source>
<translation>Veuillez entrer le mot de passe</translation>
</message>
<message>
<location filename="../src/ui/set-repo-password-dialog.cpp" line="61"/>
<source>Incorrect password</source>
<translation>Mot de passe incorrect</translation>
</message>
<message>
<location filename="../src/ui/set-repo-password-dialog.cpp" line="63"/>
<source>Unknown error</source>
<translation>Erreur inconnue</translation>
</message>
<message>
<location filename="../ui_set-repo-password-dialog.h" line="115"/>
<source>Dialog</source>
<translation>Dialogue</translation>
</message>
<message>
<location filename="../ui_set-repo-password-dialog.h" line="116"/>
<source>OK</source>
<translation>OK</translation>
</message>
<message>
<location filename="../ui_set-repo-password-dialog.h" line="117"/>
<source>Cancel</source>
<translation>Annuler</translation>
</message>
</context>
<context>
<name>SettingsDialog</name>
<message>
<location filename="../src/ui/settings-dialog.cpp" line="22"/>
<source>Settings</source>
<translation>Paramètres</translation>
</message>
<message>
<location filename="../ui_settings-dialog.h" line="217"/>
<source>Dialog</source>
<translation>Dialogue</translation>
</message>
<message>
<location filename="../ui_settings-dialog.h" line="218"/>
<source>Hide main window when started</source>
<translation>Cacher la fenêtre principale au démarrage </translation>
</message>
<message>
<location filename="../ui_settings-dialog.h" line="219"/>
<source>Notify when libraries are synchronized</source>
<translation>Notifier quand les bibliothèques sont synchronisées</translation>
</message>
<message>
<location filename="../ui_settings-dialog.h" line="220"/>
<source>Enable sync temporary files of MSOffice/Libreoffice</source>
<translation>Activer la synchronisation des fichiers temporaires MSOffice/LibreOffice</translation>
</message>
<message>
<location filename="../ui_settings-dialog.h" line="221"/>
<source>Auto start Seafile after login</source>
<translation>Démarrage automatique de Seafile après la connexion</translation>
</message>
<message>
<location filename="../ui_settings-dialog.h" line="222"/>
<source>Hide Seafile Icon from the docker</source>
<translation>Cacher l'icone Seafile de la barre</translation>
</message>
<message>
<location filename="../ui_settings-dialog.h" line="223"/>
<source>Check for new version on startup</source>
<translation>Vérifier les nouvelles versions au démarrage</translation>
</message>
<message>
<location filename="../ui_settings-dialog.h" line="224"/>
<source>Download speed limit (KB/s):</source>
<translation>Vitesse de téléchargement maximum (Ko/s) :</translation>
</message>
<message>
<location filename="../ui_settings-dialog.h" line="225"/>
<source>Upload speed limit (KB/s):</source>
<translation>Vitesse d'envoi maximum (Ko/s) :</translation>
</message>
<message>
<location filename="../ui_settings-dialog.h" line="226"/>
<source>Basic</source>
<translation>Basique</translation>
</message>
<message>
<location filename="../ui_settings-dialog.h" line="227"/>
<source>Do not automatically unsync a library</source>
<translation>Ne pas désynchroniser automatiquement une bibliothèque</translation>
</message>
<message>
<location filename="../ui_settings-dialog.h" line="228"/>
<source>Do not automatically unsync a library when its local directory is removed or unaccessible for other reasons.</source>
<translation>Ne pas désynchroniser automatiquement une bibliothèque quand le dossier local est supprimé ou inaccessible pour d'autres raisons</translation>
</message>
<message>
<location filename="../ui_settings-dialog.h" line="229"/>
<source>Do not unsync a library when not found on server</source>
<translation>Ne pas désynchroniser une bibliothèque non trouvée sur le serveur</translation>
</message>
<message>
<location filename="../ui_settings-dialog.h" line="230"/>
<source>Do not automatically unsync a library when it's not found on server</source>
<translation>Ne pas désynchroniser automatiquement une bibliothèque quand elle n'est pas trouvée sur le serveur</translation>
</message>
<message>
<location filename="../ui_settings-dialog.h" line="231"/>
<source>Enable file syncing with HTTP protocol</source>
<translation>Activer la synchronisation des fichiers avec le protocole HTTP</translation>
</message>
<message>
<location filename="../ui_settings-dialog.h" line="232"/>
<source>Do not verify server certificate in HTTP syncing</source>
<translation>Ne pas vérifier le certificat serveur lors de la synchronisation HTTP</translation>
</message>
<message>
<location filename="../ui_settings-dialog.h" line="233"/>
<source>Advanced</source>
<translation>Avancé</translation>
</message>
<message>
<location filename="../ui_settings-dialog.h" line="234"/>
<source>OK</source>
<translation>OK</translation>
</message>
<message>
<location filename="../ui_settings-dialog.h" line="235"/>
<source>Cancel</source>
<translation>Annuler</translation>
</message>
</context>
<context>
<name>SharedLinkDialog</name>
<message>
<location filename="../src/filebrowser/sharedlink-dialog.cpp" line="9"/>
<source>Share Link</source>
<translation>Lien de partage</translation>
</message>
<message>
<location filename="../src/filebrowser/sharedlink-dialog.cpp" line="12"/>
<source>Share link:</source>
<translation>Lien de partage:</translation>
</message>
<message>
<location filename="../src/filebrowser/sharedlink-dialog.cpp" line="33"/>
<source>Copy to clipboard</source>
<translation>Copier dans le presse-papiers</translation>
</message>
<message>
<location filename="../src/filebrowser/sharedlink-dialog.cpp" line="37"/>
<source>Ok</source>
<translation>Ok</translation>
</message>
</context>
<context>
<name>SslConfirmDialog</name>
<message>
<location filename="../src/ui/ssl-confirm-dialog.cpp" line="13"/>
<source>Untrusted Connection</source>
<translation>Connexion non certifiée</translation>
</message>
<message>
<location filename="../src/ui/ssl-confirm-dialog.cpp" line="16"/>
<source>%1 uses an invalid security certificate. The connection may be insecure. Do you want to continue?</source>
<translation>%1 utilise un certificat de sécurité invalide. La connexion peut ne pas être sécurisée. Voulez-vous continuer ?</translation>
</message>
<message>
<location filename="../src/ui/ssl-confirm-dialog.cpp" line="19"/>
<source>Current RSA key fingerprint is %1</source>
<translation>L'empreinte courante de la clé RSA est %1</translation>
</message>
<message>
<location filename="../src/ui/ssl-confirm-dialog.cpp" line="22"/>
<source>Previous RSA key fingerprint is %1</source>
<translation>L'empreinte précédente de la clé RSA est %1</translation>
</message>
<message>
<location filename="../ui_ssl-confirm-dialog.h" line="96"/>
<source>Dialog</source>
<translation>Dialogue</translation>
</message>
<message>
<location filename="../ui_ssl-confirm-dialog.h" line="97"/>
<source>Remember my choice</source>
<translation>Se souvenir de mon choix</translation>
</message>
<message>
<location filename="../ui_ssl-confirm-dialog.h" line="98"/>
<source>Yes</source>
<translation>Oui</translation>
</message>
<message>
<location filename="../ui_ssl-confirm-dialog.h" line="99"/>
<source>No</source>
<translation>Non</translation>
</message>
</context>
<context>
<name>StarredFilesListView</name>
<message>
<location filename="../src/ui/starred-files-list-view.cpp" line="36"/>
<source>&Open</source>
<translation>&Ouvrir</translation>
</message>
<message>
<location filename="../src/ui/starred-files-list-view.cpp" line="39"/>
<source>Open this file</source>
<translation>&Ouvrir ce fichier</translation>
</message>
<message>
<location filename="../src/ui/starred-files-list-view.cpp" line="45"/>
<source>view on &Web</source>
<translation>Voir sur &Internet</translation>
</message>
<message>
<location filename="../src/ui/starred-files-list-view.cpp" line="48"/>
<source>view this file on website</source>
<translation>voir ce fichier sur le site web</translation>
</message>
</context>
<context>
<name>StarredFilesTab</name>
<message>
<location filename="../src/ui/starred-files-tab.cpp" line="79"/>
<source>retry</source>
<translation>réessayer </translation>
</message>
<message>
<location filename="../src/ui/starred-files-tab.cpp" line="80"/>
<source>Failed to get starred files information<br/>Please %1</source>
<translation>Impossible de récupérer les informations des fichiers favoris<br/>Veuillez %1</translation>
</message>
<message>
<location filename="../src/ui/starred-files-tab.cpp" line="101"/>
<source>You have no starred files yet.</source>
<translation>Vous n'avez pas de fichiers favoris pour l'instant.</translation>
</message>
</context>
<context>
<name>TransferManager</name>
<message>
<location filename="../src/filebrowser/transfer-mgr.cpp" line="97"/>
<source>pending</source>
<translation>en attente</translation>
</message>
</context>
<context>
<name>UninstallHelperDialog</name>
<message>
<location filename="../src/ui/uninstall-helper-dialog.cpp" line="14"/>
<source>Uninstall %1</source>
<translation>Désinstallation de %1</translation>
</message>
<message>
<location filename="../src/ui/uninstall-helper-dialog.cpp" line="17"/>
<source>Do you want to remove the %1 account information?</source>
<translation>Voulez vous supprimer les informations du compte %1?</translation>
</message>
<message>
<location filename="../src/ui/uninstall-helper-dialog.cpp" line="42"/>
<source>Removing account information...</source>
<translation>Suppression des informations du compte...</translation>
</message>
<message>
<location filename="../ui_uninstall-helper-dialog.h" line="106"/>
<source>Dialog</source>
<translation>Dialogue</translation>
</message>
<message>
<location filename="../ui_uninstall-helper-dialog.h" line="107"/>
<source>text</source>
<translation>texte</translation>
</message>
<message>
<location filename="../ui_uninstall-helper-dialog.h" line="108"/>
<source>Yes</source>
<translation>Oui</translation>
</message>
<message>
<location filename="../ui_uninstall-helper-dialog.h" line="109"/>
<source>No</source>
<translation>Non</translation>
</message>
</context>
</TS> | {
"content_hash": "5f267b96e0b9410c92a9c23ba88b5c6b",
"timestamp": "",
"source": "github",
"line_count": 2455,
"max_line_length": 194,
"avg_line_length": 41.357637474541754,
"alnum_prop": 0.6347886893916264,
"repo_name": "ggkitsas/seafile-client",
"id": "1767d27cb1d8de98b38fde827f893fadef7e1287",
"size": "101970",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "i18n/seafile_fr_FR.ts",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "C",
"bytes": "3028"
},
{
"name": "C++",
"bytes": "668396"
},
{
"name": "CSS",
"bytes": "9223"
},
{
"name": "Objective-C++",
"bytes": "6272"
},
{
"name": "Python",
"bytes": "9828"
},
{
"name": "Shell",
"bytes": "649"
}
],
"symlink_target": ""
} |
/*!
* FileInput <_LANG_> Translations
*
* This file must be loaded after 'fileinput.js'. Patterns in braces '{}', or
* any HTML markup tags in the messages must not be converted or translated.
*
* @see http://github.com/kartik-v/bootstrap-fileinput
*
* NOTE: this file must be saved in UTF-8 encoding.
*/
(function ($) {
"use strict";
$.fn.fileinputLocales['_LANG_'] = {
fileSingle: 'file',
filePlural: 'files',
browseLabel: 'Browse …',
removeLabel: 'Remove',
removeTitle: 'Clear selected files',
cancelLabel: 'Cancel',
cancelTitle: 'Abort ongoing upload',
uploadLabel: 'Upload',
uploadTitle: 'Upload selected files',
msgZoomTitle: 'View details',
msgZoomModalHeading: 'Detailed Preview',
msgSizeTooLarge: 'File "{name}" (<b>{size} KB</b>) exceeds maximum allowed upload size of <b>{maxSize} KB</b>.',
msgFilesTooLess: 'You must select at least <b>{n}</b> {files} to upload.',
msgFilesTooMany: 'Number of files selected for upload <b>({n})</b> exceeds maximum allowed limit of <b>{m}</b>.',
msgFileNotFound: 'File "{name}" not found!',
msgFileSecured: 'Security restrictions prevent reading the file "{name}".',
msgFileNotReadable: 'File "{name}" is not readable.',
msgFilePreviewAborted: 'File preview aborted for "{name}".',
msgFilePreviewError: 'An error occurred while reading the file "{name}".',
msgInvalidFileType: 'Invalid type for file "{name}". Only "{types}" files are supported.',
msgInvalidFileExtension: 'Invalid extension for file "{name}". Only "{extensions}" files are supported.',
msgValidationError: 'File Upload Error',
msgLoading: 'Loading file {index} of {files} …',
msgProgress: 'Loading file {index} of {files} - {name} - {percent}% completed.',
msgSelected: '{n} {files} selected',
msgFoldersNotAllowed: 'Drag & drop files only! Skipped {n} dropped folder(s).',
msgImageWidthSmall: 'Width of image file "{name}" must be at least {size} px.',
msgImageHeightSmall: 'Height of image file "{name}" must be at least {size} px.',
msgImageWidthLarge: 'Width of image file "{name}" cannot exceed {size} px.',
msgImageHeightLarge: 'Height of image file "{name}" cannot exceed {size} px.',
dropZoneTitle: 'Drag & drop files here …',
fileActionSettings: {
removeTitle: 'Remove file',
uploadTitle: 'Upload file',
indicatorNewTitle: 'Not uploaded yet',
indicatorSuccessTitle: 'Uploaded',
indicatorErrorTitle: 'Upload Error',
indicatorLoadingTitle: 'Uploading ...'
}
};
})(window.jQuery); | {
"content_hash": "8011a1d79018902dce7db0104079e4fd",
"timestamp": "",
"source": "github",
"line_count": 55,
"max_line_length": 121,
"avg_line_length": 50.50909090909091,
"alnum_prop": 0.6321094312455003,
"repo_name": "Cinquefoil/Population-Health-Dashboard",
"id": "6c302f7cffb2a0f0c1252f8a85b4291586acd691",
"size": "2778",
"binary": false,
"copies": "2",
"ref": "refs/heads/gh-pages",
"path": "js/fileinput_locale_LANG.js",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "ApacheConf",
"bytes": "169"
},
{
"name": "CSS",
"bytes": "140265"
},
{
"name": "HTML",
"bytes": "1312"
},
{
"name": "JavaScript",
"bytes": "1383684"
},
{
"name": "Nginx",
"bytes": "379"
},
{
"name": "PHP",
"bytes": "3589680"
}
],
"symlink_target": ""
} |
Author: "José F. Romaniello"
Description: "Dark theme inspired by Obsidian"
FileName: "GrandsonOfObsidian.tmTheme"
ID: "GrandsonOfObsidian"
Title: "Grandson of Obsidian"
layout: theme
---
| {
"content_hash": "12cca77c4c65d545544fb068977685c1",
"timestamp": "",
"source": "github",
"line_count": 8,
"max_line_length": 48,
"avg_line_length": 25.25,
"alnum_prop": 0.7227722772277227,
"repo_name": "Colorsublime/colorsublime.github.io",
"id": "01958d2439ec1878d3bf2576be566b1782edd2f3",
"size": "207",
"binary": false,
"copies": "1",
"ref": "refs/heads/dev",
"path": "_themes/Grandson of Obsidian.md",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "ABAP",
"bytes": "1037"
},
{
"name": "ActionScript",
"bytes": "1133"
},
{
"name": "Ada",
"bytes": "99"
},
{
"name": "Assembly",
"bytes": "506"
},
{
"name": "AutoHotkey",
"bytes": "720"
},
{
"name": "Batchfile",
"bytes": "260"
},
{
"name": "C#",
"bytes": "83"
},
{
"name": "C++",
"bytes": "808"
},
{
"name": "COBOL",
"bytes": "4"
},
{
"name": "CSS",
"bytes": "1048800"
},
{
"name": "Cirru",
"bytes": "520"
},
{
"name": "Clojure",
"bytes": "794"
},
{
"name": "CoffeeScript",
"bytes": "403"
},
{
"name": "ColdFusion",
"bytes": "86"
},
{
"name": "Common Lisp",
"bytes": "632"
},
{
"name": "D",
"bytes": "324"
},
{
"name": "Dart",
"bytes": "489"
},
{
"name": "Dockerfile",
"bytes": "2493"
},
{
"name": "Eiffel",
"bytes": "375"
},
{
"name": "Elixir",
"bytes": "692"
},
{
"name": "Elm",
"bytes": "487"
},
{
"name": "Erlang",
"bytes": "487"
},
{
"name": "Forth",
"bytes": "979"
},
{
"name": "Fortran",
"bytes": "713"
},
{
"name": "FreeMarker",
"bytes": "1017"
},
{
"name": "G-code",
"bytes": "521"
},
{
"name": "GLSL",
"bytes": "512"
},
{
"name": "Gherkin",
"bytes": "699"
},
{
"name": "Go",
"bytes": "641"
},
{
"name": "Groovy",
"bytes": "1080"
},
{
"name": "HTML",
"bytes": "1288890"
},
{
"name": "Haskell",
"bytes": "512"
},
{
"name": "Haxe",
"bytes": "447"
},
{
"name": "Io",
"bytes": "140"
},
{
"name": "JSONiq",
"bytes": "4"
},
{
"name": "Java",
"bytes": "1550"
},
{
"name": "JavaScript",
"bytes": "13412104"
},
{
"name": "Julia",
"bytes": "210"
},
{
"name": "Kotlin",
"bytes": "3556"
},
{
"name": "LSL",
"bytes": "2080"
},
{
"name": "Liquid",
"bytes": "1883"
},
{
"name": "LiveScript",
"bytes": "5747"
},
{
"name": "Lua",
"bytes": "981"
},
{
"name": "MATLAB",
"bytes": "203"
},
{
"name": "Makefile",
"bytes": "6612"
},
{
"name": "Mask",
"bytes": "597"
},
{
"name": "NSIS",
"bytes": "486"
},
{
"name": "Nix",
"bytes": "2212"
},
{
"name": "OCaml",
"bytes": "539"
},
{
"name": "Objective-C",
"bytes": "2672"
},
{
"name": "OpenSCAD",
"bytes": "333"
},
{
"name": "PHP",
"bytes": "351"
},
{
"name": "PLpgSQL",
"bytes": "2547"
},
{
"name": "Pascal",
"bytes": "1412"
},
{
"name": "Perl",
"bytes": "678"
},
{
"name": "PigLatin",
"bytes": "277"
},
{
"name": "PowerShell",
"bytes": "418"
},
{
"name": "Python",
"bytes": "478"
},
{
"name": "R",
"bytes": "2445"
},
{
"name": "Ruby",
"bytes": "2208"
},
{
"name": "Rust",
"bytes": "495"
},
{
"name": "Scala",
"bytes": "1541"
},
{
"name": "Scheme",
"bytes": "559"
},
{
"name": "Shell",
"bytes": "4256"
},
{
"name": "Swift",
"bytes": "476"
},
{
"name": "Tcl",
"bytes": "899"
},
{
"name": "TeX",
"bytes": "1345"
},
{
"name": "TypeScript",
"bytes": "1672"
},
{
"name": "VBScript",
"bytes": "938"
},
{
"name": "VHDL",
"bytes": "830"
},
{
"name": "Vala",
"bytes": "485"
},
{
"name": "Verilog",
"bytes": "274"
},
{
"name": "Vim Snippet",
"bytes": "228243"
},
{
"name": "Wollok",
"bytes": "419"
},
{
"name": "XQuery",
"bytes": "114"
},
{
"name": "Zeek",
"bytes": "689"
}
],
"symlink_target": ""
} |
package com.pigtimer.timer;
import com.pigtimer.service.ActivitiesModeService;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Component;
import javax.annotation.Resource;
/**
* Created by tosy on 2017/3/24.
*/
@Component
public class ActivitiesModeTimer {
private final Logger logger = LoggerFactory.getLogger(this.getClass());
@Resource
ActivitiesModeService am;
@Scheduled(cron="0 0/1 * * * ?") //每分钟执行一次
public void statusCheck() {
logger.info("每分钟执行一次。开始……");
logger.info("每分钟执行一次。结束。");
}
@Scheduled(fixedRate=10000)
public void testTasks10() {
logger.info("每7秒执行一次。开始……");
am.showKeys("6381");
am.insertdbtest();
logger.info("每7秒执行一次。结束。");
}
@Scheduled(fixedRate=5000)
public void testTasks5() {
logger.info("每5秒执行一次。开始……");
am.showKeys("6380");
am.getdball();
logger.info("每5秒执行一次。结束。");
}
}
| {
"content_hash": "b923527eee48b2647a37f73df98ca5db",
"timestamp": "",
"source": "github",
"line_count": 45,
"max_line_length": 75,
"avg_line_length": 23.288888888888888,
"alnum_prop": 0.6545801526717557,
"repo_name": "tosy2015/springdemo",
"id": "d0ab92b2366886bc8d28044384902b810c1e8b5f",
"size": "1192",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "src/main/java/com/pigtimer/timer/ActivitiesModeTimer.java",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "Java",
"bytes": "18380"
}
],
"symlink_target": ""
} |
'use strict';
var bodyParser = require('body-parser');
var path = require('path');
var mongoose = require('mongoose');
var express = require('express');
var app = express();
mongoose.Promise = global.Promise;
mongoose.connect('mongodb://localhost/dog_dashboard');
var DogSchema = new mongoose.Schema({
name: String,
age: Number,
height: Number
});
mongoose.model('Dog', DogSchema);
var Dog = mongoose.model('Dog')
app.use(express.static(path.join(__dirname, '/static')));
app.use(bodyParser.urlencoded({extended: true}));
app.set('/views', path.join(__dirname, '/views'));
app.set('view engine', 'ejs');
app.get('/', function(request, response) {
/* find all Dogs in db */
Dog.find({}, function(errors, dogs) {
if (errors) {
console.log('/: errors finding all users in db...');
for (var index in errors) {
console.log('-', errors[index]);
}
response.redirect('/');
} else {
response.render('index', {dogs: dogs});
}
});
});
app.get('/dogs/view/:id', function(request, response) {
Dog.findOne({_id: request.params.id}, function(errors, dog) {
if(errors) {
console.log('/dogs/view/id: errors occured during find of document...');
for (var index in errors) {
console.log('-', errors[index]);
}
} else {
response.render('view', { dog: dog });
}
});
});
app.get('/dogs/edit/:id', function(request, response) {
Dog.findOne({_id: request.params.id}, function(errors, dog) {
if(errors) {
console.log('/dogs/edit/id: errors occured during find of document...');
for (var index in errors) {
console.log('-', errors[index]);
}
} else {
response.render('edit', { dog: dog });
}
});
});
app.get('/dogs/remove/:id', function(request, response) {
Dog.remove({_id: request.params.id}, function(errors) {
if (errors) {
console.log('/dogs/remove/id: errors occured during removal of document...');
for (var index in errors) {
console.log('-', errors[index]);
}
} else {
response.redirect('/');
}
});
});
app.post('/dogs/add', function(request, response) {
if (!request.body.name || !request.body.age || !request.body.height) {
console.log('/dogs/add: missing necessary fields from post data');
response.redirect('/');
return;
}
var dog = new Dog({name: request.body.name, age: request.body.age, height: request.body.height});
dog.save(function(errors, dog) {
if(errors) {
console.log('/dogs/add: errors occured during save of document...');
for (var index in errors) {
console.log('-', errors[index]);
}
} else {
response.redirect('/');
}
});
});
app.post('/dogs/update/:id', function(request, response) {
if (!request.body.name || !request.body.age || !request.body.height) {
console.log('/dogs/add: missing necessary fields from post data');
response.redirect('/');
return;
}
Dog.update({_id: request.params.id}, {$set: {name: request.body.name, age: request.body.age, height: request.body.height}}, function(errors) {
if(errors) {
console.log('/dogs/update/id: errors occured during update of document...');
for (var index in errors) {
console.log('-', errors[index]);
}
} else {
response.redirect('/');
}
});
});
app.listen(8000, function() {
console.log('listening on port 8000...');
}); | {
"content_hash": "1f72780a421a3326fe1a593b8efeb7b2",
"timestamp": "",
"source": "github",
"line_count": 129,
"max_line_length": 143,
"avg_line_length": 25.496124031007753,
"alnum_prop": 0.6284584980237155,
"repo_name": "franciscojt/nice",
"id": "c3ca2b5d2b9e4f89c3fcc84db0a66e1c97c89340",
"size": "3289",
"binary": false,
"copies": "3",
"ref": "refs/heads/master",
"path": "Woodall_Robert/Assignments/mongodb_mongoose/mongoose_dashboard/server.js",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "CSS",
"bytes": "3487"
},
{
"name": "HTML",
"bytes": "240607"
},
{
"name": "JavaScript",
"bytes": "343501"
},
{
"name": "Python",
"bytes": "836"
}
],
"symlink_target": ""
} |
import { IController, IComponentOptions, copy, module, toJson } from 'angular';
import { cloneDeep } from 'lodash';
import { ApplicationWriter } from 'core/application/service/ApplicationWriter';
import { Application } from 'core/application/application.model';
import './configSectionFooter.component.less';
export interface IConfigSectionFooterViewState {
originalConfig: any;
originalStringVal: string;
saving: boolean;
saveError: boolean;
isDirty: boolean;
}
export class ConfigSectionFooterController implements IController {
public viewState: IConfigSectionFooterViewState;
public application: Application;
public config: any;
public configField: string;
public saveDisabled: boolean;
public revert(): void {
copy(this.viewState.originalConfig, this.config);
this.viewState.isDirty = false;
}
private saveSuccess(): void {
this.viewState.originalConfig = cloneDeep(this.config);
this.viewState.originalStringVal = toJson(this.config);
this.viewState.isDirty = false;
this.viewState.saving = false;
this.application.attributes[this.configField] = this.config;
}
private saveError(): void {
this.viewState.saving = false;
this.viewState.saveError = true;
}
public save(): void {
this.viewState.saving = true;
this.viewState.saveError = false;
const updateCommand: any = {
name: this.application.name,
accounts: this.application.attributes.accounts,
};
updateCommand[this.configField] = this.config;
ApplicationWriter.updateApplication(updateCommand).then(
() => this.saveSuccess(),
() => this.saveError(),
);
}
}
const configSectionFooterComponent: IComponentOptions = {
bindings: {
application: '=',
config: '=',
viewState: '=',
configField: '@',
revert: '&?',
afterSave: '&?',
saveDisabled: '<',
},
controller: ConfigSectionFooterController,
templateUrl: require('./configSectionFooter.component.html'),
};
export const CONFIG_SECTION_FOOTER = 'spinnaker.core.application.config.section.footer.component';
module(CONFIG_SECTION_FOOTER, []).component('configSectionFooter', configSectionFooterComponent);
| {
"content_hash": "d75e67f70c9ab03919936bf822ebee77",
"timestamp": "",
"source": "github",
"line_count": 75,
"max_line_length": 98,
"avg_line_length": 29.106666666666666,
"alnum_prop": 0.7191937700412276,
"repo_name": "sgarlick987/deck",
"id": "ba4b7fdf03c3a0285d92b82937203839fe65604a",
"size": "2183",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "app/scripts/modules/core/src/application/config/footer/configSectionFooter.component.ts",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "CSS",
"bytes": "188919"
},
{
"name": "HTML",
"bytes": "1284008"
},
{
"name": "JavaScript",
"bytes": "2004278"
},
{
"name": "Shell",
"bytes": "24704"
},
{
"name": "TypeScript",
"bytes": "5219772"
}
],
"symlink_target": ""
} |
import os
from ievv_opensource.utils.ievvbuildstatic import pluginbase
from ievv_opensource.utils.ievvbuildstatic.filepath import AbstractDjangoAppPath
from ievv_opensource.utils.shellcommandmixin import ShellCommandError
from ievv_opensource.utils.shellcommandmixin import ShellCommandMixin
class Plugin(pluginbase.Plugin, ShellCommandMixin):
"""
Browserify javascript bundler plugin.
Examples:
Simple example::
IEVVTASKS_BUILDSTATIC_APPS = ievvbuildstatic.config.Apps(
ievvbuildstatic.config.App(
appname='demoapp',
version='1.0.0',
plugins=[
ievvbuildstatic.browserify_jsbuild.Plugin(
sourcefile='app.js',
destinationfile='app.js',
),
]
)
)
Custom source folder example::
IEVVTASKS_BUILDSTATIC_APPS = ievvbuildstatic.config.Apps(
ievvbuildstatic.config.App(
appname='demoapp',
version='1.0.0',
plugins=[
ievvbuildstatic.browserify_jsbuild.Plugin(
sourcefolder=os.path.join('scripts', 'javascript', 'api'),
sourcefile='api.js',
destinationfile='api.js',
),
]
)
)
"""
name = 'browserify_jsbuild'
default_group = 'js'
def __init__(self, sourcefile, destinationfile,
sourcefolder=os.path.join('scripts', 'javascript'),
destinationfolder=os.path.join('scripts'),
extra_watchfolders=None,
extra_import_paths=None,
sourcemap=True,
**kwargs):
"""
Parameters:
sourcefile: The source file relative to ``sourcefolder``.
destinationfile: Path to destination file relative to ``destinationfolder``.
sourcefolder: The folder where ``sourcefiles`` is located relative to
the source folder of the :class:`~ievv_opensource.utils.ievvbuild.config.App`.
Defaults to ``scripts/javascript``.
destinationfolder: The folder where ``destinationfile`` is located relative to
the destination folder of the :class:`~ievv_opensource.utils.ievvbuild.config.App`.
Defaults to ``scripts/``.
extra_watchfolders: List of extra folders to watch for changes.
Relative to the source folder of the
:class:`~ievv_opensource.utils.ievvbuild.config.App`.
**kwargs: Kwargs for :class:`ievv_opensource.utils.ievvbuildstatic.pluginbase.Plugin`.
"""
super(Plugin, self).__init__(**kwargs)
self.sourcefile = sourcefile
self.destinationfile = destinationfile
self.destinationfolder = destinationfolder
self.sourcefolder = sourcefolder
self.extra_watchfolders = extra_watchfolders or []
self.sourcemap = sourcemap
self.extra_import_paths = extra_import_paths or []
def get_sourcefile_path(self):
return self.app.get_source_path(self.sourcefolder, self.sourcefile)
def get_destinationfile_path(self):
return self.app.get_destination_path(self.destinationfolder, self.destinationfile)
def get_default_import_paths(self):
return ['node_modules']
def get_import_paths(self):
return self.get_default_import_paths() + self.extra_import_paths
def _get_import_paths_as_strlist(self):
import_paths = []
for path in self.get_import_paths():
if isinstance(path, AbstractDjangoAppPath):
path = path.abspath
import_paths.append(path)
return import_paths
def install(self):
"""
Installs the ``browserify`` NPM package.
The package is installed with no version specified, so you
probably want to freeze the version using the
:class:`ievv_opensource.utils.ievvbuildstatic.npminstall.Plugin` plugin.
"""
self.app.get_installer('npm').queue_install(
'browserify', installtype='dev')
if self.app.apps.is_in_production_mode():
self.app.get_installer('npm').queue_install(
'uglifyify', installtype='dev')
def get_browserify_executable(self):
return self.app.get_installer('npm').find_executable('browserify')
def get_browserify_extra_args(self):
"""
Get extra browserify args.
Override this in subclasses to add transforms and such.
"""
return []
def get_browserify_production_args(self):
return [
'-t', '[', 'uglifyify', ']',
'-g', 'uglifyify',
]
def make_browserify_args(self):
"""
Make browserify args list.
Adds the following in the listed order:
- ``-d`` if the ``sourcemap`` kwarg is ``True``.
- the source file.
- ``-d <destination file path>``.
- whatever :meth:`.get_browserify_extra_args` returns.
Should normally not be extended. Extend :meth:`.get_browserify_extra_args`
instead.
"""
args = []
if self.sourcemap:
args.append('-d')
args.extend([
self.get_sourcefile_path(),
'-o', self.get_destinationfile_path(),
])
args.extend(self.get_browserify_extra_args())
if self.app.apps.is_in_production_mode():
args.extend(self.get_browserify_production_args())
return args
def make_browserify_executable_environment(self):
environment = os.environ.copy()
environment.update({
'NODE_PATH': ':'.join(self._get_import_paths_as_strlist())
})
return environment
def post_run(self):
"""
Called at the start of run(), after the initial command start logmessage,
but before any other code is executed.
Does nothing by default, but subclasses can hook in
configuration code here if they need to generate config
files, perform validation of file structure, etc.
"""
def run(self):
self.get_logger().command_start(
'Running browserify with {sourcefile} as input and {destinationfile} as output'.format(
sourcefile=self.get_sourcefile_path(),
destinationfile=self.get_destinationfile_path()))
self.post_run()
executable = self.get_browserify_executable()
destination_folder = self.app.get_destination_path(self.destinationfolder)
if not os.path.exists(destination_folder):
os.makedirs(destination_folder)
try:
self.run_shell_command(executable, args=self.make_browserify_args(),
_cwd=self.app.get_source_path(),
_env=self.make_browserify_executable_environment())
except ShellCommandError:
self.get_logger().command_error('browserify build FAILED!')
else:
self.get_logger().command_success('browserify build succeeded :)')
def get_extra_watchfolder_paths(self):
return map(self.app.get_source_path, self.extra_watchfolders)
def get_watch_folders(self):
"""
We only watch the folder where the javascript is located,
so this returns the absolute path of the ``sourcefolder``.
"""
folders = [self.app.get_source_path(self.sourcefolder)]
if self.extra_watchfolders:
folders.extend(self.get_extra_watchfolder_paths())
return folders
def get_watch_extensions(self):
"""
Returns a list of the extensions to watch for in watch mode.
Defaults to ``['js']``.
Unless you have complex needs, it is probably easier to override
this than overriding
:meth:`~ievv_opensource.utils.ievvbuildstatic.pluginbase.Plugin.get_watch_regexes`.
"""
return ['js']
def get_watch_regexes(self):
return ['^.+[.]({extensions})'.format(
extensions='|'.join(self.get_watch_extensions()))]
def __str__(self):
return '{}({})'.format(super(Plugin, self).__str__(), self.sourcefolder)
| {
"content_hash": "ebc49ee482f97d75f3630bdb2e2e8c06",
"timestamp": "",
"source": "github",
"line_count": 223,
"max_line_length": 99,
"avg_line_length": 37.9237668161435,
"alnum_prop": 0.5887430530921131,
"repo_name": "appressoas/ievv_opensource",
"id": "ea1411aa0cfb935a211e3c3053ced358a1ceb22f",
"size": "8457",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "ievv_opensource/utils/ievvbuildstatic/browserify_jsbuild.py",
"mode": "33188",
"license": "bsd-3-clause",
"language": [
{
"name": "CoffeeScript",
"bytes": "199"
},
{
"name": "Dockerfile",
"bytes": "162"
},
{
"name": "HTML",
"bytes": "7544"
},
{
"name": "JavaScript",
"bytes": "719"
},
{
"name": "Less",
"bytes": "27"
},
{
"name": "Python",
"bytes": "614046"
},
{
"name": "SCSS",
"bytes": "199"
},
{
"name": "Shell",
"bytes": "141"
},
{
"name": "TypeScript",
"bytes": "254"
}
],
"symlink_target": ""
} |
from flask_wtf import FlaskForm
from wtforms import *
from wtforms.validators import DataRequired
from werkzeug.datastructures import MultiDict
from app.main.models.Item_cardapio import Item_cardapio_dao
class modal_item_cardapio(FlaskForm):
item_cardapio = SelectField('item_cardapio')
item = ''
quantidade = IntegerField("quatidade", validators=[DataRequired()])
lista_item_exta =[]
def reset(self):
blankData = MultiDict([('csrf_token', self.reset)])
self.process(blankData)
| {
"content_hash": "cc4648bad710016c236c7e6f3c2dc35e",
"timestamp": "",
"source": "github",
"line_count": 19,
"max_line_length": 71,
"avg_line_length": 27.36842105263158,
"alnum_prop": 0.7269230769230769,
"repo_name": "amandapersampa/MicroGerencia",
"id": "21467086f2c4d8b8cbf72f863d34cd815cbc4bd1",
"size": "520",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "app/main/forms/modal_item_cardapio.py",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "CSS",
"bytes": "308099"
},
{
"name": "Gherkin",
"bytes": "1268"
},
{
"name": "HTML",
"bytes": "32893"
},
{
"name": "Mako",
"bytes": "494"
},
{
"name": "Python",
"bytes": "35799"
}
],
"symlink_target": ""
} |
<h1>{{title}}</h1>
<div class="container">
<div class="row">
<div class="col-sm-12">
<br>
</div>
<div class="col-sm-6">
<form class="animated bounceIn" (ngSubmit)="crearUsuario(NuevoUsuarioForm)" #NuevoUsuarioForm="ngForm">
<div class="form-group">
<label>Usuario</label>
<input required
minlength="4"
type="text"
class="form-control"
placeholder="Digite su nombre"
name="nombre"
[(ngModel)]="nuevoUsuario.nombre"
#nombre="ngModel"
#nombreElm>
</div>
<div class="form-group">
<label>Ciudad Residencia</label>
<input required
type="text"
class="form-control"
placeholder="Digite su ciudad de Residencia actual"
name="ciudadResidencia"
[(ngModel)]="nuevoUsuario.ciudadResidencia"
#ciudadResidencia="ngModel"
#nombreElm>
</div>
<div class="form-group">
<label>Fecha Nacimiento</label>
<input type="date"
class="form-control"
placeholder="Ingrese su fecha de nacimiento"
name="fechaNacimiento"
[(ngModel)]="nuevoUsuario.fechaNacimiento"
#fechaNacimiento="ngModel"
#nombreElm>
</div>
<button [disabled]="disabledButtons.NuevoUsuarioFormSubmitButton||!NuevoUsuarioForm.valid" type="submit"
class="btn btn-block btn-success">Crear
</button>
</form>
</div>
<br>
</div>
<div class="row">
<div class="col-sm-12 animated flipInX" *ngFor="let usuario of usuarios">
<div class="text-left">
<h3>{{usuario.nombre}}</h3>
<p>ID: {{usuario.id}}</p>
</div>
<div class="row animated flipInY" [hidden]="!usuario.FormularioCerrado">
<div class="col-sm-5">
<button class="btn btn-block btn-info"
(click)="usuario.FormularioCerrado = !usuario.FormularioCerrado"
>Actualizar
</button>
</div>
<div class="col-sm-2"></div>
<div class="col-sm-5">
<button class="btn btn-block btn-danger" (click)="borrarUsuario(usuario.id)">Borrar</button>
</div>
<div class="col-sm-12">
<a [routerLink]="[usuario.id,'borrachera']">Ir a las Borracheras de {{usuario.nombre}}...</a>
</div>
</div>
<div class="div" [hidden]="usuario.FormularioCerrado">
<form action="">
<form class="animated bounceIn" (ngSubmit)="actualizarUsuario(usuario)" #EditFormUsuario="ngForm">
<div class="form-group">
<label>Nombre</label>
<input required
type="text"
class="form-control"
placeholder="Digite un nombre de tienda como: Adidas"
name="nombre"
[(ngModel)]="usuario.nombre"
#nombre="ngModel"
#nombreElm>
</div>
<div class="form-group">
<label>Ciudad Residencia</label>
<input required
type="text"
class="form-control"
placeholder="Digite su ciudad de Residencia actual"
name="ciudadResidencia"
[(ngModel)]="usuario.ciudadResidencia"
#ciudadResidencia="ngModel"
#nombreElm>
</div>
<div class="form-group">
<label>Fecha Nacimiento</label>
<input type="date"
class="form-control"
placeholder="Ingrese su fecha de nacimiento"
name="fechaNacimiento"
[(ngModel)]="usuario.fechaNacimiento"
#fechaNacimiento="ngModel"
#nombreElm>
</div>
<button [disabled]="disabledButtons.EditBorracheraForm||!EditFormUsuario.valid" type="submit"
class="btn btn-block btn-success">Update
</button>
<button type="button"
class="btn btn-block btn-warning"
(click)="usuario.FormularioCerrado = !usuario.FormularioCerrado"
>
Cancelar
</button>
</form>
</form>
</div>
</div>
</div>
</div>
| {
"content_hash": "7ec863c50e51c2c5908af9d43cf2b4dc",
"timestamp": "",
"source": "github",
"line_count": 126,
"max_line_length": 112,
"avg_line_length": 36.357142857142854,
"alnum_prop": 0.49727133813577823,
"repo_name": "PatricioAlejandro/examen-twj-chavezpatricio",
"id": "e1bbbe635c5cba8979bed6a0db37ecea83335082",
"size": "4581",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "frontBorrachera/src/app/usuario/usuario.component.html",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "CSS",
"bytes": "80"
},
{
"name": "HTML",
"bytes": "96787"
},
{
"name": "JavaScript",
"bytes": "3512660"
},
{
"name": "TypeScript",
"bytes": "16822"
}
],
"symlink_target": ""
} |
import sys
import json5_generator
import make_runtime_features
import name_utilities
import template_expander
# We want exactly the same parsing as RuntimeFeatureWriter
# but generate different files.
class OriginTrialsWriter(make_runtime_features.BaseRuntimeFeatureWriter):
file_basename = 'origin_trials'
def __init__(self, json5_file_path, output_dir):
super(OriginTrialsWriter, self).__init__(json5_file_path, output_dir)
self._outputs = {
(self.file_basename + '.cc'): self.generate_implementation,
}
self._implied_mappings = self._make_implied_mappings()
self._trial_to_features_map = self._make_trial_to_features_map()
self._max_features_per_trial = max(
len(features) for features in self._trial_to_features_map.values())
self._set_trial_types()
@property
def origin_trial_features(self):
return self._origin_trial_features
def _make_implied_mappings(self):
# Set up the implied_by relationships between trials.
implied_mappings = dict()
for implied_feature in (feature
for feature in self._origin_trial_features
if feature['origin_trial_feature_name']
and feature['implied_by']):
# An origin trial can only be implied by other features that also
# have a trial defined.
implied_by_trials = []
for implied_by_name in implied_feature['implied_by']:
if any(implied_by_name == feature['name'].original
and feature['origin_trial_feature_name']
for feature in self._origin_trial_features):
implied_by_trials.append(implied_by_name)
# Keep a list of origin trial features implied for each
# trial. This is essentially an inverse of the implied_by
# list attached to each feature.
implied_list = implied_mappings.get(implied_by_name)
if implied_list is None:
implied_list = set()
implied_mappings[implied_by_name] = implied_list
implied_list.add(implied_feature['name'].original)
implied_feature['implied_by_origin_trials'] = implied_by_trials
return implied_mappings
def _make_trial_to_features_map(self):
trial_feature_mappings = {}
for feature in [
feature for feature in self._origin_trial_features
if feature['origin_trial_feature_name']
]:
trial_name = feature['origin_trial_feature_name']
if trial_name in trial_feature_mappings:
trial_feature_mappings[trial_name].append(feature)
else:
trial_feature_mappings[trial_name] = [feature]
return trial_feature_mappings
def _set_trial_types(self):
for feature in self._origin_trial_features:
trial_type = feature['origin_trial_type']
if feature[
'origin_trial_allows_insecure'] and trial_type != 'deprecation':
raise Exception('Origin trial must have type deprecation to '
'specify origin_trial_allows_insecure: %s' %
feature['name'])
if trial_type:
feature[
'origin_trial_type'] = name_utilities._upper_camel_case(
trial_type)
@template_expander.use_jinja('templates/' + file_basename + '.cc.tmpl')
def generate_implementation(self):
return {
'features': self._features,
'origin_trial_features': self._origin_trial_features,
'implied_origin_trial_features': self._implied_mappings,
'trial_to_features_map': self._trial_to_features_map,
'max_features_per_trial': self._max_features_per_trial,
'input_files': self._input_files,
}
if __name__ == '__main__':
json5_generator.Maker(OriginTrialsWriter).main()
| {
"content_hash": "45006935b89596264fe82c1f1c07ffef",
"timestamp": "",
"source": "github",
"line_count": 98,
"max_line_length": 84,
"avg_line_length": 42.46938775510204,
"alnum_prop": 0.58097068716963,
"repo_name": "endlessm/chromium-browser",
"id": "db5b93a998e28fcaa8ec63a52fc4e2229de671db",
"size": "5714",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "third_party/blink/renderer/build/scripts/make_origin_trials.py",
"mode": "33261",
"license": "bsd-3-clause",
"language": [],
"symlink_target": ""
} |
<?xml version="1.0" encoding="ascii"?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en" lang="en">
<head>
<title>gluon.html.COLGROUP</title>
<link rel="stylesheet" href="epydoc.css" type="text/css" />
<script type="text/javascript" src="epydoc.js"></script>
</head>
<body bgcolor="white" text="black" link="blue" vlink="#204080"
alink="#204080">
<!-- ==================== NAVIGATION BAR ==================== -->
<table class="navbar" border="0" width="100%" cellpadding="0"
bgcolor="#a0c0ff" cellspacing="0">
<tr valign="middle">
<!-- Home link -->
<th> <a
href="gluon-module.html">Home</a> </th>
<!-- Tree link -->
<th> <a
href="module-tree.html">Trees</a> </th>
<!-- Index link -->
<th> <a
href="identifier-index.html">Indices</a> </th>
<!-- Help link -->
<th> <a
href="help.html">Help</a> </th>
<!-- Project homepage -->
<th class="navbar" align="right" width="100%">
<table border="0" cellpadding="0" cellspacing="0">
<tr><th class="navbar" align="center"
><a class="navbar" target="_top" href="http://www.web2py.com">web2py Web Framework</a></th>
</tr></table></th>
</tr>
</table>
<table width="100%" cellpadding="0" cellspacing="0">
<tr valign="top">
<td width="100%">
<span class="breadcrumbs">
<a href="gluon-module.html">Package gluon</a> ::
<a href="gluon.html-module.html" onclick="show_private();">Module html</a> ::
Class COLGROUP
</span>
</td>
<td>
<table cellpadding="0" cellspacing="0">
<!-- hide/show private -->
<tr><td align="right"><span class="options">[<a href="javascript:void(0);" class="privatelink"
onclick="toggle_private();">hide private</a>]</span></td></tr>
<tr><td align="right"><span class="options"
>[<a href="frames.html" target="_top">frames</a
>] | <a href="gluon.html.COLGROUP-class.html"
target="_top">no frames</a>]</span></td></tr>
</table>
</td>
</tr>
</table>
<!-- ==================== CLASS DESCRIPTION ==================== -->
<h1 class="epydoc">Class COLGROUP</h1><p class="nomargin-top"><span class="codelink"><a href="gluon.html-pysrc.html#COLGROUP">source code</a></span></p>
<pre class="base-tree">
object --+
|
<a href="gluon.html.XmlComponent-class.html" onclick="show_private();">XmlComponent</a> --+
|
<a href="gluon.html.DIV-class.html">DIV</a> --+
|
<strong class="uidshort">COLGROUP</strong>
</pre>
<hr />
<!-- ==================== INSTANCE METHODS ==================== -->
<a name="section-InstanceMethods"></a>
<table class="summary" border="1" cellpadding="3"
cellspacing="0" width="100%" bgcolor="white">
<tr bgcolor="#70b0f0" class="table-header">
<td colspan="2" class="table-header">
<table border="0" cellpadding="0" cellspacing="0" width="100%">
<tr valign="top">
<td align="left"><span class="table-header">Instance Methods</span></td>
<td align="right" valign="top"
><span class="options">[<a href="#section-InstanceMethods"
class="privatelink" onclick="toggle_private();"
>hide private</a>]</span></td>
</tr>
</table>
</td>
</tr>
<tr>
<td colspan="2" class="summary">
<p class="indent-wrapped-lines"><b>Inherited from <code><a href="gluon.html.DIV-class.html">DIV</a></code></b>:
<code><a href="gluon.html.DIV-class.html#__delitem__">__delitem__</a></code>,
<code><a href="gluon.html.DIV-class.html#__getitem__">__getitem__</a></code>,
<code><a href="gluon.html.DIV-class.html#__init__">__init__</a></code>,
<code><a href="gluon.html.DIV-class.html#__len__">__len__</a></code>,
<code><a href="gluon.html.DIV-class.html#__nonzero__">__nonzero__</a></code>,
<code><a href="gluon.html.DIV-class.html#__setitem__">__setitem__</a></code>,
<code><a href="gluon.html.DIV-class.html#__str__">__str__</a></code>,
<code><a href="gluon.html.DIV-class.html#append">append</a></code>,
<code><a href="gluon.html.DIV-class.html#element">element</a></code>,
<code><a href="gluon.html.DIV-class.html#elements">elements</a></code>,
<code><a href="gluon.html.DIV-class.html#flatten">flatten</a></code>,
<code><a href="gluon.html.DIV-class.html#insert">insert</a></code>,
<code><a href="gluon.html.DIV-class.html#sibling">sibling</a></code>,
<code><a href="gluon.html.DIV-class.html#siblings">siblings</a></code>,
<code><a href="gluon.html.DIV-class.html#update">update</a></code>,
<code><a href="gluon.html.DIV-class.html#xml">xml</a></code>
</p>
<div class="private"> <p class="indent-wrapped-lines"><b>Inherited from <code><a href="gluon.html.DIV-class.html">DIV</a></code></b> (private):
<code><a href="gluon.html.DIV-class.html#_fixup" onclick="show_private();">_fixup</a></code>,
<code><a href="gluon.html.DIV-class.html#_postprocessing" onclick="show_private();">_postprocessing</a></code>,
<code><a href="gluon.html.DIV-class.html#_setnode" onclick="show_private();">_setnode</a></code>,
<code><a href="gluon.html.DIV-class.html#_traverse" onclick="show_private();">_traverse</a></code>,
<code><a href="gluon.html.DIV-class.html#_validate" onclick="show_private();">_validate</a></code>,
<code><a href="gluon.html.DIV-class.html#_wrap_components" onclick="show_private();">_wrap_components</a></code>,
<code><a href="gluon.html.DIV-class.html#_xml" onclick="show_private();">_xml</a></code>
</p></div>
<p class="indent-wrapped-lines"><b>Inherited from <code><a href="gluon.html.XmlComponent-class.html" onclick="show_private();">XmlComponent</a></code></b>:
<code><a href="gluon.html.XmlComponent-class.html#__add__">__add__</a></code>,
<code><a href="gluon.html.XmlComponent-class.html#__mul__">__mul__</a></code>,
<code><a href="gluon.html.XmlComponent-class.html#add_class">add_class</a></code>,
<code><a href="gluon.html.XmlComponent-class.html#remove_class">remove_class</a></code>
</p>
<p class="indent-wrapped-lines"><b>Inherited from <code>object</code></b>:
<code>__delattr__</code>,
<code>__format__</code>,
<code>__getattribute__</code>,
<code>__hash__</code>,
<code>__new__</code>,
<code>__reduce__</code>,
<code>__reduce_ex__</code>,
<code>__repr__</code>,
<code>__setattr__</code>,
<code>__sizeof__</code>,
<code>__subclasshook__</code>
</p>
</td>
</tr>
</table>
<!-- ==================== CLASS VARIABLES ==================== -->
<a name="section-ClassVariables"></a>
<table class="summary" border="1" cellpadding="3"
cellspacing="0" width="100%" bgcolor="white">
<tr bgcolor="#70b0f0" class="table-header">
<td colspan="2" class="table-header">
<table border="0" cellpadding="0" cellspacing="0" width="100%">
<tr valign="top">
<td align="left"><span class="table-header">Class Variables</span></td>
<td align="right" valign="top"
><span class="options">[<a href="#section-ClassVariables"
class="privatelink" onclick="toggle_private();"
>hide private</a>]</span></td>
</tr>
</table>
</td>
</tr>
<tr>
<td width="15%" align="right" valign="top" class="summary">
<span class="summary-type"> </span>
</td><td class="summary">
<a name="tag"></a><span class="summary-name">tag</span> = <code title="'colgroup'"><code class="variable-quote">'</code><code class="variable-string">colgroup</code><code class="variable-quote">'</code></code>
</td>
</tr>
<tr>
<td colspan="2" class="summary">
<p class="indent-wrapped-lines"><b>Inherited from <code><a href="gluon.html.DIV-class.html">DIV</a></code></b>:
<code><a href="gluon.html.DIV-class.html#regex_attr">regex_attr</a></code>,
<code><a href="gluon.html.DIV-class.html#regex_class">regex_class</a></code>,
<code><a href="gluon.html.DIV-class.html#regex_id">regex_id</a></code>,
<code><a href="gluon.html.DIV-class.html#regex_tag">regex_tag</a></code>
</p>
</td>
</tr>
</table>
<!-- ==================== PROPERTIES ==================== -->
<a name="section-Properties"></a>
<table class="summary" border="1" cellpadding="3"
cellspacing="0" width="100%" bgcolor="white">
<tr bgcolor="#70b0f0" class="table-header">
<td colspan="2" class="table-header">
<table border="0" cellpadding="0" cellspacing="0" width="100%">
<tr valign="top">
<td align="left"><span class="table-header">Properties</span></td>
<td align="right" valign="top"
><span class="options">[<a href="#section-Properties"
class="privatelink" onclick="toggle_private();"
>hide private</a>]</span></td>
</tr>
</table>
</td>
</tr>
<tr>
<td colspan="2" class="summary">
<p class="indent-wrapped-lines"><b>Inherited from <code>object</code></b>:
<code>__class__</code>
</p>
</td>
</tr>
</table>
<!-- ==================== NAVIGATION BAR ==================== -->
<table class="navbar" border="0" width="100%" cellpadding="0"
bgcolor="#a0c0ff" cellspacing="0">
<tr valign="middle">
<!-- Home link -->
<th> <a
href="gluon-module.html">Home</a> </th>
<!-- Tree link -->
<th> <a
href="module-tree.html">Trees</a> </th>
<!-- Index link -->
<th> <a
href="identifier-index.html">Indices</a> </th>
<!-- Help link -->
<th> <a
href="help.html">Help</a> </th>
<!-- Project homepage -->
<th class="navbar" align="right" width="100%">
<table border="0" cellpadding="0" cellspacing="0">
<tr><th class="navbar" align="center"
><a class="navbar" target="_top" href="http://www.web2py.com">web2py Web Framework</a></th>
</tr></table></th>
</tr>
</table>
<table border="0" cellpadding="0" cellspacing="0" width="100%%">
<tr>
<td align="left" class="footer">
Generated by Epydoc 3.0.1 on Thu Nov 28 13:54:41 2013
</td>
<td align="right" class="footer">
<a target="mainFrame" href="http://epydoc.sourceforge.net"
>http://epydoc.sourceforge.net</a>
</td>
</tr>
</table>
<script type="text/javascript">
<!--
// Private objects are initially displayed (because if
// javascript is turned off then we want them to be
// visible); but by default, we want to hide them. So hide
// them unless we have a cookie that says to show them.
checkCookie();
// -->
</script>
</body>
</html>
| {
"content_hash": "f0ee9eb14ea9796bf673ce164a59b51c",
"timestamp": "",
"source": "github",
"line_count": 254,
"max_line_length": 217,
"avg_line_length": 43.76377952755905,
"alnum_prop": 0.5775458798128823,
"repo_name": "elrafael/web2py-test",
"id": "904ba262b702fcef4dc43d0857c972af7fb946a1",
"size": "11116",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "applications/examples/static/epydoc/gluon.html.COLGROUP-class.html",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "CSS",
"bytes": "159727"
},
{
"name": "JavaScript",
"bytes": "1402927"
},
{
"name": "LiveScript",
"bytes": "6103"
},
{
"name": "Python",
"bytes": "1019962"
},
{
"name": "Shell",
"bytes": "91020"
},
{
"name": "Tcl",
"bytes": "2287768"
}
],
"symlink_target": ""
} |
@import UIKit;
@interface SAAppDelegate : UIResponder <UIApplicationDelegate>
@property (strong, nonatomic) UIWindow *window;
@end
| {
"content_hash": "e575c8c4a6633e67dca9803850278798",
"timestamp": "",
"source": "github",
"line_count": 7,
"max_line_length": 62,
"avg_line_length": 19.142857142857142,
"alnum_prop": 0.7835820895522388,
"repo_name": "logansease/SeaseAssist",
"id": "7d30d7d385c73ee69c439e9f8e1e834cbc8c4e03",
"size": "279",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "Example/SeaseAssist/SAAppDelegate.h",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "C",
"bytes": "319"
},
{
"name": "Objective-C",
"bytes": "261340"
},
{
"name": "Ruby",
"bytes": "1974"
},
{
"name": "Shell",
"bytes": "35"
},
{
"name": "Swift",
"bytes": "16041"
}
],
"symlink_target": ""
} |
<!doctype html>
<title>CodeMirror: Clojure mode</title>
<meta charset="utf-8"/>
<link rel=stylesheet href="../../doc/docs.css">
<link rel="stylesheet" href="../../lib/codemirror.css">
<script src="../../lib/codemirror.js"></script>
<script src="clojure.js"></script>
<style>.CodeMirror {
background: #f8f8f8;
}</style>
<div id=nav>
<a href="http://codemirror.net"><h1>CodeMirror</h1><img id=logo src="../../doc/logo.png"></a>
<ul>
<li><a href="../../index.html">Home</a>
<li><a href="../../doc/manual.html">Manual</a>
<li><a href="https://github.com/codemirror/codemirror">Code</a>
</ul>
<ul>
<li><a href="../index.html">Language modes</a>
<li><a class=active href="#">Clojure</a>
</ul>
</div>
<article>
<h2>Clojure mode</h2>
<form><textarea id="code" name="code">
; Conway's Game of Life, based on the work of:
;; Laurent Petit https://gist.github.com/1200343
;; Christophe Grand http://clj-me.cgrand.net/2011/08/19/conways-game-of-life
(ns ^{:doc "Conway's Game of Life."}
game-of-life)
;; Core game of life's algorithm functions
(defn neighbours
"Given a cell's coordinates, returns the coordinates of its neighbours."
[[x y]]
(for [dx [-1 0 1] dy (if (zero? dx) [-1 1] [-1 0 1])]
[(+ dx x) (+ dy y)]))
(defn step
"Given a set of living cells, computes the new set of living cells."
[cells]
(set (for [[cell n] (frequencies (mapcat neighbours cells))
:when (or (= n 3) (and (= n 2) (cells cell)))]
cell)))
;; Utility methods for displaying game on a text terminal
(defn print-board
"Prints a board on *out*, representing a step in the game."
[board w h]
(doseq [x (range (inc w)) y (range (inc h))]
(if (= y 0) (print "\n"))
(print (if (board [x y]) "[X]" " . "))))
(defn display-grids
"Prints a squence of boards on *out*, representing several steps."
[grids w h]
(doseq [board grids]
(print-board board w h)
(print "\n")))
;; Launches an example board
(def
^{:doc "board represents the initial set of living cells"}
board #{[2 1] [2 2] [2 3]})
(display-grids (take 3 (iterate step board)) 5 5)
;; Let's play with characters
(println \1 \a \# \\
\" \( \newline
\} \" \space
\tab \return \backspace
\u1000 \uAaAa \u9F9F)
</textarea></form>
<script>
var editor = CodeMirror.fromTextArea(document.getElementById("code"), {});
</script>
<p><strong>MIME types defined:</strong> <code>text/x-clojure</code>.</p>
</article>
| {
"content_hash": "bdab839d24c5c183fa2e427f6fbc27a6",
"timestamp": "",
"source": "github",
"line_count": 91,
"max_line_length": 97,
"avg_line_length": 27.912087912087912,
"alnum_prop": 0.5992125984251968,
"repo_name": "NCSSM-CS/CSAssess",
"id": "618305e98a99bd788473937a3f36a22231e786c9",
"size": "2540",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "view/codemirror/mode/clojure/index.html",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "CSS",
"bytes": "11074"
},
{
"name": "HTML",
"bytes": "602701"
},
{
"name": "JavaScript",
"bytes": "308272"
},
{
"name": "Python",
"bytes": "107267"
},
{
"name": "Shell",
"bytes": "291"
}
],
"symlink_target": ""
} |
from decimal import *
import getpass
import math
import os
import os.path
import platform
import sys
import time
from jsonrpc import ServiceProxy, json
BASE_FEE=Decimal("0.001")
def check_json_precision():
"""Make sure json library being used does not lose precision converting BTC values"""
n = Decimal("20000000.00000003")
satoshis = int(json.loads(json.dumps(float(n)))*1.0e8)
if satoshis != 2000000000000003:
raise RuntimeError("JSON encode/decode loses precision")
def determine_db_dir():
"""Return the default location of the eternity data directory"""
if platform.system() == "Darwin":
return os.path.expanduser("~/Library/Application Support/Eternity/")
elif platform.system() == "Windows":
return os.path.join(os.environ['APPDATA'], "Eternity")
return os.path.expanduser("~/.eternity")
def read_bitcoin_config(dbdir):
"""Read the eternity.conf file from dbdir, returns dictionary of settings"""
from ConfigParser import SafeConfigParser
class FakeSecHead(object):
def __init__(self, fp):
self.fp = fp
self.sechead = '[all]\n'
def readline(self):
if self.sechead:
try: return self.sechead
finally: self.sechead = None
else:
s = self.fp.readline()
if s.find('#') != -1:
s = s[0:s.find('#')].strip() +"\n"
return s
config_parser = SafeConfigParser()
config_parser.readfp(FakeSecHead(open(os.path.join(dbdir, "eternity.conf"))))
return dict(config_parser.items("all"))
def connect_JSON(config):
"""Connect to a eternity JSON-RPC server"""
testnet = config.get('testnet', '0')
testnet = (int(testnet) > 0) # 0/1 in config file, convert to True/False
if not 'rpcport' in config:
config['rpcport'] = 14854 if testnet else 4854
connect = "http://%s:%[email protected]:%s"%(config['rpcuser'], config['rpcpassword'], config['rpcport'])
try:
result = ServiceProxy(connect)
# ServiceProxy is lazy-connect, so send an RPC command mostly to catch connection errors,
# but also make sure the eternityd we're talking to is/isn't testnet:
if result.getmininginfo()['testnet'] != testnet:
sys.stderr.write("RPC server at "+connect+" testnet setting mismatch\n")
sys.exit(1)
return result
except:
sys.stderr.write("Error connecting to RPC server at "+connect+"\n")
sys.exit(1)
def unlock_wallet(eternityd):
info = eternityd.getinfo()
if 'unlocked_until' not in info:
return True # wallet is not encrypted
t = int(info['unlocked_until'])
if t <= time.time():
try:
passphrase = getpass.getpass("Wallet is locked; enter passphrase: ")
eternityd.walletpassphrase(passphrase, 5)
except:
sys.stderr.write("Wrong passphrase\n")
info = eternityd.getinfo()
return int(info['unlocked_until']) > time.time()
def list_available(eternityd):
address_summary = dict()
address_to_account = dict()
for info in eternityd.listreceivedbyaddress(0):
address_to_account[info["address"]] = info["account"]
unspent = eternityd.listunspent(0)
for output in unspent:
# listunspent doesn't give addresses, so:
rawtx = eternityd.getrawtransaction(output['txid'], 1)
vout = rawtx["vout"][output['vout']]
pk = vout["scriptPubKey"]
# This code only deals with ordinary pay-to-eternity-address
# or pay-to-script-hash outputs right now; anything exotic is ignored.
if pk["type"] != "pubkeyhash" and pk["type"] != "scripthash":
continue
address = pk["addresses"][0]
if address in address_summary:
address_summary[address]["total"] += vout["value"]
address_summary[address]["outputs"].append(output)
else:
address_summary[address] = {
"total" : vout["value"],
"outputs" : [output],
"account" : address_to_account.get(address, "")
}
return address_summary
def select_coins(needed, inputs):
# Feel free to improve this, this is good enough for my simple needs:
outputs = []
have = Decimal("0.0")
n = 0
while have < needed and n < len(inputs):
outputs.append({ "txid":inputs[n]["txid"], "vout":inputs[n]["vout"]})
have += inputs[n]["amount"]
n += 1
return (outputs, have-needed)
def create_tx(eternityd, fromaddresses, toaddress, amount, fee):
all_coins = list_available(eternityd)
total_available = Decimal("0.0")
needed = amount+fee
potential_inputs = []
for addr in fromaddresses:
if addr not in all_coins:
continue
potential_inputs.extend(all_coins[addr]["outputs"])
total_available += all_coins[addr]["total"]
if total_available < needed:
sys.stderr.write("Error, only %f BTC available, need %f\n"%(total_available, needed));
sys.exit(1)
#
# Note:
# Python's json/jsonrpc modules have inconsistent support for Decimal numbers.
# Instead of wrestling with getting json.dumps() (used by jsonrpc) to encode
# Decimals, I'm casting amounts to float before sending them to eternityd.
#
outputs = { toaddress : float(amount) }
(inputs, change_amount) = select_coins(needed, potential_inputs)
if change_amount > BASE_FEE: # don't bother with zero or tiny change
change_address = fromaddresses[-1]
if change_address in outputs:
outputs[change_address] += float(change_amount)
else:
outputs[change_address] = float(change_amount)
rawtx = eternityd.createrawtransaction(inputs, outputs)
signed_rawtx = eternityd.signrawtransaction(rawtx)
if not signed_rawtx["complete"]:
sys.stderr.write("signrawtransaction failed\n")
sys.exit(1)
txdata = signed_rawtx["hex"]
return txdata
def compute_amount_in(eternityd, txinfo):
result = Decimal("0.0")
for vin in txinfo['vin']:
in_info = eternityd.getrawtransaction(vin['txid'], 1)
vout = in_info['vout'][vin['vout']]
result = result + vout['value']
return result
def compute_amount_out(txinfo):
result = Decimal("0.0")
for vout in txinfo['vout']:
result = result + vout['value']
return result
def sanity_test_fee(eternityd, txdata_hex, max_fee):
class FeeError(RuntimeError):
pass
try:
txinfo = eternityd.decoderawtransaction(txdata_hex)
total_in = compute_amount_in(eternityd, txinfo)
total_out = compute_amount_out(txinfo)
if total_in-total_out > max_fee:
raise FeeError("Rejecting transaction, unreasonable fee of "+str(total_in-total_out))
tx_size = len(txdata_hex)/2
kb = tx_size/1000 # integer division rounds down
if kb > 1 and fee < BASE_FEE:
raise FeeError("Rejecting no-fee transaction, larger than 1000 bytes")
if total_in < 0.01 and fee < BASE_FEE:
raise FeeError("Rejecting no-fee, tiny-amount transaction")
# Exercise for the reader: compute transaction priority, and
# warn if this is a very-low-priority transaction
except FeeError as err:
sys.stderr.write((str(err)+"\n"))
sys.exit(1)
def main():
import optparse
parser = optparse.OptionParser(usage="%prog [options]")
parser.add_option("--from", dest="fromaddresses", default=None,
help="addresses to get eternitys from")
parser.add_option("--to", dest="to", default=None,
help="address to get send eternitys to")
parser.add_option("--amount", dest="amount", default=None,
help="amount to send")
parser.add_option("--fee", dest="fee", default="0.0",
help="fee to include")
parser.add_option("--datadir", dest="datadir", default=determine_db_dir(),
help="location of eternity.conf file with RPC username/password (default: %default)")
parser.add_option("--testnet", dest="testnet", default=False, action="store_true",
help="Use the test network")
parser.add_option("--dry_run", dest="dry_run", default=False, action="store_true",
help="Don't broadcast the transaction, just create and print the transaction data")
(options, args) = parser.parse_args()
check_json_precision()
config = read_bitcoin_config(options.datadir)
if options.testnet: config['testnet'] = True
eternityd = connect_JSON(config)
if options.amount is None:
address_summary = list_available(eternityd)
for address,info in address_summary.iteritems():
n_transactions = len(info['outputs'])
if n_transactions > 1:
print("%s %.8f %s (%d transactions)"%(address, info['total'], info['account'], n_transactions))
else:
print("%s %.8f %s"%(address, info['total'], info['account']))
else:
fee = Decimal(options.fee)
amount = Decimal(options.amount)
while unlock_wallet(eternityd) == False:
pass # Keep asking for passphrase until they get it right
txdata = create_tx(eternityd, options.fromaddresses.split(","), options.to, amount, fee)
sanity_test_fee(eternityd, txdata, amount*Decimal("0.01"))
if options.dry_run:
print(txdata)
else:
txid = eternityd.sendrawtransaction(txdata)
print(txid)
if __name__ == '__main__':
main()
| {
"content_hash": "d8004255ac8c95492b8561c676f593ff",
"timestamp": "",
"source": "github",
"line_count": 252,
"max_line_length": 111,
"avg_line_length": 38.49603174603175,
"alnum_prop": 0.6175651994639728,
"repo_name": "baltcoinh/eternity",
"id": "ee31b282d1552452323a3ffeedc490129eb33dc7",
"size": "10082",
"binary": false,
"copies": "1",
"ref": "refs/heads/patch-2",
"path": "contrib/spendfrom/spendfrom.py",
"mode": "33261",
"license": "mit",
"language": [
{
"name": "Assembly",
"bytes": "7639"
},
{
"name": "C",
"bytes": "989736"
},
{
"name": "C++",
"bytes": "4188197"
},
{
"name": "CSS",
"bytes": "39853"
},
{
"name": "HTML",
"bytes": "50621"
},
{
"name": "Java",
"bytes": "2100"
},
{
"name": "M4",
"bytes": "141745"
},
{
"name": "Makefile",
"bytes": "87715"
},
{
"name": "Objective-C",
"bytes": "3922"
},
{
"name": "Objective-C++",
"bytes": "7243"
},
{
"name": "Protocol Buffer",
"bytes": "2308"
},
{
"name": "Python",
"bytes": "211442"
},
{
"name": "QMake",
"bytes": "25681"
},
{
"name": "Roff",
"bytes": "18058"
},
{
"name": "Shell",
"bytes": "45318"
}
],
"symlink_target": ""
} |
// Copyright 2019 The Chromium Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package org.chromium.chrome.browser.tasks.tab_management;
import static org.junit.Assert.assertEquals;
import static org.mockito.ArgumentMatchers.any;
import static org.mockito.ArgumentMatchers.eq;
import static org.mockito.Mockito.doReturn;
import static org.mockito.Mockito.verify;
import static org.chromium.chrome.browser.tasks.tab_management.suggestions.TabSuggestionFeedback.TabSuggestionResponse.ACCEPTED;
import static org.chromium.chrome.browser.tasks.tab_management.suggestions.TabSuggestionFeedback.TabSuggestionResponse.DISMISSED;
import static org.chromium.chrome.browser.tasks.tab_management.suggestions.TabSuggestionFeedback.TabSuggestionResponse.NOT_CONSIDERED;
import android.content.Context;
import org.junit.Before;
import org.junit.Rule;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.mockito.ArgumentCaptor;
import org.mockito.Captor;
import org.mockito.Mock;
import org.mockito.MockitoAnnotations;
import org.chromium.base.Callback;
import org.chromium.base.test.util.JniMocker;
import org.chromium.chrome.browser.profiles.Profile;
import org.chromium.chrome.browser.profiles.ProfileJni;
import org.chromium.chrome.browser.tab.Tab;
import org.chromium.chrome.browser.tabmodel.TabModel;
import org.chromium.chrome.browser.tabmodel.TabModelFilterProvider;
import org.chromium.chrome.browser.tabmodel.TabModelSelector;
import org.chromium.chrome.browser.tasks.tab_groups.TabGroupModelFilter;
import org.chromium.chrome.browser.tasks.tab_management.suggestions.TabContext;
import org.chromium.chrome.browser.tasks.tab_management.suggestions.TabSuggestion;
import org.chromium.chrome.browser.tasks.tab_management.suggestions.TabSuggestionFeedback;
import org.chromium.testing.local.LocalRobolectricTestRunner;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
/**
* Unit tests for {@link TabSuggestionMessageService}.
*/
@SuppressWarnings({"ResultOfMethodCallIgnored", "ArraysAsListWithZeroOrOneArgument"})
@RunWith(LocalRobolectricTestRunner.class)
public class TabSuggestionMessageServiceUnitTest {
private static final int TAB1_ID = 456;
private static final int TAB2_ID = 789;
private static final int TAB3_ID = 123;
private static final int POSITION1 = 0;
private static final int POSITION2 = 1;
private static final int POSITION3 = 2;
private static final int TAB1_ROOT_ID = TAB1_ID;
private static final int TAB2_ROOT_ID = TAB2_ID;
private static final int TAB3_ROOT_ID = TAB2_ID;
private static final int CLOSE_SUGGESTION_ACTION_BUTTON_RESOURCE_ID =
org.chromium.chrome.tab_ui.R.string.tab_suggestion_close_tab_action_button;
private static final int GROUP_SUGGESTION_ACTION_BUTTON_RESOURCE_ID =
org.chromium.chrome.tab_ui.R.string.tab_selection_editor_group;
private Tab mTab1;
private Tab mTab2;
private Tab mTab3;
private TabSuggestionMessageService mMessageService;
@Rule
public JniMocker mocker = new JniMocker();
@Mock
public Profile.Natives mMockProfileNatives;
@Mock
Context mContext;
@Mock
TabModelSelector mTabModelSelector;
@Mock
TabModel mTabModel;
@Mock
TabModelFilterProvider mTabModelFilterProvider;
@Mock
TabGroupModelFilter mTabGroupModelFilter;
@Mock
TabSelectionEditorCoordinator.TabSelectionEditorController mTabSelectionEditorController;
@Mock
Callback<TabSuggestionFeedback> mTabSuggestionFeedbackCallback;
@Captor
ArgumentCaptor<TabSuggestionFeedback> mTabSuggestionFeedbackCallbackArgumentCaptor;
@Before
public void setUp() {
// After setUp there are three tabs in TabModel.
MockitoAnnotations.initMocks(this);
mocker.mock(ProfileJni.TEST_HOOKS, mMockProfileNatives);
// Set up Tabs.
mTab1 = TabUiUnitTestUtils.prepareTab(TAB1_ID, TAB1_ROOT_ID, "");
mTab2 = TabUiUnitTestUtils.prepareTab(TAB2_ID, TAB2_ROOT_ID, "");
mTab3 = TabUiUnitTestUtils.prepareTab(TAB3_ID, TAB3_ROOT_ID, "");
// Set up TabModelSelector.
doReturn(mTabModel).when(mTabModelSelector).getCurrentModel();
doReturn(mTab1).when(mTabModelSelector).getTabById(TAB1_ID);
doReturn(mTab2).when(mTabModelSelector).getTabById(TAB2_ID);
doReturn(mTab3).when(mTabModelSelector).getTabById(TAB3_ID);
// Set up TabModel.
doReturn(3).when(mTabModel).getCount();
doReturn(mTab1).when(mTabModel).getTabAt(POSITION1);
doReturn(mTab2).when(mTabModel).getTabAt(POSITION2);
doReturn(mTab3).when(mTabModel).getTabAt(POSITION3);
// Set up TabModelFilter.
doReturn(mTabModelFilterProvider).when(mTabModelSelector).getTabModelFilterProvider();
doReturn(mTabGroupModelFilter).when(mTabModelFilterProvider).getCurrentTabModelFilter();
doReturn(mTabModel).when(mTabGroupModelFilter).getTabModel();
mMessageService = new TabSuggestionMessageService(
mContext, mTabModelSelector, mTabSelectionEditorController);
}
// Tests for Close suggestions.
@Test
public void testReviewHandler_closeSuggestion() {
prepareTabSuggestions(Arrays.asList(mTab1, mTab2), TabSuggestion.TabSuggestionAction.CLOSE);
String closeSuggestionActionButtonText = "close";
int expectedEnablingThreshold =
TabSuggestionMessageService.CLOSE_SUGGESTION_ACTION_ENABLING_THRESHOLD;
doReturn(closeSuggestionActionButtonText)
.when(mContext)
.getString(eq(CLOSE_SUGGESTION_ACTION_BUTTON_RESOURCE_ID));
mMessageService.review();
verify(mTabSelectionEditorController)
.configureToolbar(eq(closeSuggestionActionButtonText), any(),
eq(expectedEnablingThreshold), any());
verify(mTabSelectionEditorController).show(eq(Arrays.asList(mTab1, mTab2, mTab3)), eq(2));
prepareTabSuggestions(Arrays.asList(mTab1, mTab3), TabSuggestion.TabSuggestionAction.CLOSE);
mMessageService.review();
verify(mTabSelectionEditorController).show(eq(Arrays.asList(mTab1, mTab3, mTab2)), eq(2));
}
@Test
public void testClosingSuggestionActionHandler() {
List<Tab> suggestedTabs = Arrays.asList(mTab1, mTab2);
List<Integer> suggestedTabIds = Arrays.asList(TAB1_ID, TAB2_ID);
List<TabSuggestion> tabSuggestions =
prepareTabSuggestions(suggestedTabs, TabSuggestion.TabSuggestionAction.CLOSE);
TabSuggestion bestSuggestion = tabSuggestions.get(0);
assertEquals(3, mTabModelSelector.getCurrentModel().getCount());
TabSelectionEditorActionProvider actionProvider =
mMessageService.getActionProvider(bestSuggestion);
actionProvider.processSelectedTabs(suggestedTabs, mTabModelSelector);
verify(mTabModel).closeMultipleTabs(eq(suggestedTabs), eq(true));
verify(mTabSuggestionFeedbackCallback)
.onResult(mTabSuggestionFeedbackCallbackArgumentCaptor.capture());
TabSuggestionFeedback capturedFeedback =
mTabSuggestionFeedbackCallbackArgumentCaptor.getValue();
assertEquals(bestSuggestion, capturedFeedback.tabSuggestion);
assertEquals(ACCEPTED, capturedFeedback.tabSuggestionResponse);
assertEquals(suggestedTabIds, capturedFeedback.selectedTabIds);
assertEquals(3, capturedFeedback.totalTabCount);
}
@Test
public void testClosingSuggestionNavigationHandler() {
List<Tab> suggestedTabs = Arrays.asList(mTab1, mTab2);
List<TabSuggestion> tabSuggestions =
prepareTabSuggestions(suggestedTabs, TabSuggestion.TabSuggestionAction.CLOSE);
TabSuggestion bestSuggestion = tabSuggestions.get(0);
TabSelectionEditorCoordinator.TabSelectionEditorNavigationProvider navigationProvider =
mMessageService.getNavigationProvider();
navigationProvider.goBack();
verify(mTabSuggestionFeedbackCallback)
.onResult(mTabSuggestionFeedbackCallbackArgumentCaptor.capture());
TabSuggestionFeedback capturedFeedback =
mTabSuggestionFeedbackCallbackArgumentCaptor.getValue();
assertEquals(bestSuggestion, capturedFeedback.tabSuggestion);
assertEquals(DISMISSED, capturedFeedback.tabSuggestionResponse);
}
// Tests for grouping suggestion
@Test
public void testReviewHandler_groupSuggestion() {
prepareTabSuggestions(Arrays.asList(mTab1, mTab2), TabSuggestion.TabSuggestionAction.GROUP);
String groupSuggestionActionButtonText = "group";
int expectedEnablingThreshold =
TabSuggestionMessageService.GROUP_SUGGESTION_ACTION_ENABLING_THRESHOLD;
doReturn(groupSuggestionActionButtonText)
.when(mContext)
.getString(eq(GROUP_SUGGESTION_ACTION_BUTTON_RESOURCE_ID));
mMessageService.review();
verify(mTabSelectionEditorController)
.configureToolbar(eq(groupSuggestionActionButtonText), any(),
eq(expectedEnablingThreshold), any());
verify(mTabSelectionEditorController).show(eq(Arrays.asList(mTab1, mTab2, mTab3)), eq(2));
prepareTabSuggestions(Arrays.asList(mTab1, mTab3), TabSuggestion.TabSuggestionAction.GROUP);
mMessageService.review();
verify(mTabSelectionEditorController).show(eq(Arrays.asList(mTab1, mTab3, mTab2)), eq(2));
}
@Test
public void testGroupingSuggestionActionHandler() {
List<Tab> suggestedTabs = Arrays.asList(mTab1, mTab2);
List<Integer> suggestedTabIds = Arrays.asList(TAB1_ID, TAB2_ID);
List<TabSuggestion> tabSuggestions =
prepareTabSuggestions(suggestedTabs, TabSuggestion.TabSuggestionAction.GROUP);
TabSuggestion bestSuggestion = tabSuggestions.get(0);
assertEquals(3, mTabModelSelector.getCurrentModel().getCount());
TabSelectionEditorActionProvider actionProvider =
mMessageService.getActionProvider(bestSuggestion);
actionProvider.processSelectedTabs(suggestedTabs, mTabModelSelector);
verify(mTabSuggestionFeedbackCallback)
.onResult(mTabSuggestionFeedbackCallbackArgumentCaptor.capture());
verify(mTabGroupModelFilter)
.mergeListOfTabsToGroup(eq(suggestedTabs), any(), eq(false), eq(true));
TabSuggestionFeedback capturedFeedback =
mTabSuggestionFeedbackCallbackArgumentCaptor.getValue();
assertEquals(bestSuggestion, capturedFeedback.tabSuggestion);
assertEquals(ACCEPTED, capturedFeedback.tabSuggestionResponse);
assertEquals(suggestedTabIds, capturedFeedback.selectedTabIds);
assertEquals(3, capturedFeedback.totalTabCount);
}
@Test
public void testDismiss() {
List<Tab> suggestedTabs = Arrays.asList(mTab1, mTab2);
List<TabSuggestion> tabSuggestions =
prepareTabSuggestions(suggestedTabs, TabSuggestion.TabSuggestionAction.CLOSE);
TabSuggestion bestSuggestion = tabSuggestions.get(0);
mMessageService.dismiss();
verify(mTabSuggestionFeedbackCallback)
.onResult(mTabSuggestionFeedbackCallbackArgumentCaptor.capture());
TabSuggestionFeedback capturedFeedback =
mTabSuggestionFeedbackCallbackArgumentCaptor.getValue();
assertEquals(bestSuggestion, capturedFeedback.tabSuggestion);
assertEquals(NOT_CONSIDERED, capturedFeedback.tabSuggestionResponse);
}
@Test(expected = AssertionError.class)
public void testInvalidatedSuggestion() {
mMessageService.onTabSuggestionInvalidated();
mMessageService.review();
}
private List<TabSuggestion> prepareTabSuggestions(
List<Tab> suggestedTab, @TabSuggestion.TabSuggestionAction int actionCode) {
List<TabContext.TabInfo> suggestedTabInfo = new ArrayList<>();
for (int i = 0; i < suggestedTab.size(); i++) {
TabContext.TabInfo tabInfo = TabContext.TabInfo.createFromTab(suggestedTab.get(i));
suggestedTabInfo.add(tabInfo);
}
TabSuggestion suggestion = new TabSuggestion(suggestedTabInfo, actionCode, "");
List<TabSuggestion> tabSuggestions =
Collections.unmodifiableList(Arrays.asList(suggestion));
mMessageService.onNewSuggestion(tabSuggestions, mTabSuggestionFeedbackCallback);
return tabSuggestions;
}
}
| {
"content_hash": "cb09564f3cd7c532d2bdf2685ddec632",
"timestamp": "",
"source": "github",
"line_count": 281,
"max_line_length": 134,
"avg_line_length": 45.1779359430605,
"alnum_prop": 0.7360378101614808,
"repo_name": "endlessm/chromium-browser",
"id": "6e942ccfbbd916658f057969b13dfdd91db44de1",
"size": "12695",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "chrome/android/features/tab_ui/junit/src/org/chromium/chrome/browser/tasks/tab_management/TabSuggestionMessageServiceUnitTest.java",
"mode": "33188",
"license": "bsd-3-clause",
"language": [],
"symlink_target": ""
} |
package org.apache.openwhisk.standalone
import java.io.File
import java.nio.charset.StandardCharsets.UTF_8
import akka.actor.ActorSystem
import akka.stream.ActorMaterializer
import org.apache.commons.io.{FileUtils, IOUtils}
import org.apache.openwhisk.common.{Logging, TransactionId}
import org.apache.openwhisk.standalone.StandaloneDockerSupport.{checkOrAllocatePort, containerName, createRunCmd}
import pureconfig.loadConfigOrThrow
import scala.concurrent.{ExecutionContext, Future}
class UserEventLauncher(docker: StandaloneDockerClient,
owPort: Int,
kafkaDockerPort: Int,
existingUserEventSvcPort: Option[Int],
workDir: File,
dataDir: File)(implicit logging: Logging,
ec: ExecutionContext,
actorSystem: ActorSystem,
materializer: ActorMaterializer,
tid: TransactionId) {
//owPort+1 is used by Api Gateway
private val userEventPort = existingUserEventSvcPort.getOrElse(checkOrAllocatePort(owPort + 2))
private val prometheusPort = checkOrAllocatePort(9090)
private val grafanaPort = checkOrAllocatePort(3000)
case class UserEventConfig(image: String, prometheusImage: String, grafanaImage: String)
private val userEventConfig = loadConfigOrThrow[UserEventConfig](StandaloneConfigKeys.userEventConfigKey)
private val hostIp = StandaloneDockerSupport.getLocalHostIp()
def run(): Future[Seq[ServiceContainer]] = {
for {
userEvent <- runUserEvents()
(promContainer, promSvc) <- runPrometheus()
grafanaSvc <- runGrafana(promContainer)
} yield {
logging.info(this, "Enabled the user-event config")
System.setProperty("whisk.user-events.enabled", "true")
Seq(userEvent, promSvc, grafanaSvc)
}
}
def runUserEvents(): Future[ServiceContainer] = {
existingUserEventSvcPort match {
case Some(_) =>
logging.info(this, s"Connecting to pre existing user-event service at $userEventPort")
Future.successful(ServiceContainer(userEventPort, s"http://localhost:$userEventPort", "Existing user-event"))
case None =>
val env = Map("KAFKA_HOSTS" -> s"$hostIp:$kafkaDockerPort")
logging.info(this, s"Starting User Events: $userEventPort")
val name = containerName("user-events")
val params = Map("-p" -> Set(s"$userEventPort:9095"))
val args = createRunCmd(name, env, params)
val f = docker.runDetached(userEventConfig.image, args, true)
f.map(_ => ServiceContainer(userEventPort, s"http://localhost:$userEventPort", name))
}
}
def runPrometheus(): Future[(StandaloneDockerContainer, ServiceContainer)] = {
logging.info(this, s"Starting Prometheus at $prometheusPort")
val baseParams = Map("-p" -> Set(s"$prometheusPort:9090"))
val promConfigDir = newDir(workDir, "prometheus")
val promDataDir = newDir(dataDir, "prometheus")
val configFile = new File(promConfigDir, "prometheus.yml")
FileUtils.write(configFile, prometheusConfig, UTF_8)
val volParams = Map(
"-v" -> Set(s"${promDataDir.getAbsolutePath}:/prometheus", s"${promConfigDir.getAbsolutePath}:/etc/prometheus/"))
val name = containerName("prometheus")
val args = createRunCmd(name, Map.empty, baseParams ++ volParams)
val f = docker.runDetached(userEventConfig.prometheusImage, args, shouldPull = true)
val sc = ServiceContainer(prometheusPort, s"http://localhost:$prometheusPort", name)
f.map(c => (c, sc))
}
def runGrafana(promContainer: StandaloneDockerContainer): Future[ServiceContainer] = {
logging.info(this, s"Starting Grafana at $grafanaPort")
val baseParams = Map("-p" -> Set(s"$grafanaPort:3000"))
val grafanaConfigDir = newDir(workDir, "grafana")
val grafanaDataDir = newDir(dataDir, "grafana")
val promUrl = s"http://$hostIp:$prometheusPort"
unzipGrafanaConfig(grafanaConfigDir, promUrl)
val env = Map(
"GF_PATHS_PROVISIONING" -> "/etc/grafana/provisioning",
"GF_USERS_ALLOW_SIGN_UP" -> "false",
"GF_AUTH_ANONYMOUS_ENABLED" -> "true",
"GF_AUTH_ANONYMOUS_ORG_NAME" -> "Main Org.",
"GF_AUTH_ANONYMOUS_ORG_ROLE" -> "Admin")
val volParams = Map(
"-v" -> Set(
s"${grafanaDataDir.getAbsolutePath}:/var/lib/grafana",
s"${grafanaConfigDir.getAbsolutePath}/provisioning/:/etc/grafana/provisioning/",
s"${grafanaConfigDir.getAbsolutePath}/dashboards/:/var/lib/grafana/dashboards/"))
val name = containerName("grafana")
val args = createRunCmd(name, env, baseParams ++ volParams)
val f = docker.runDetached(userEventConfig.grafanaImage, args, shouldPull = true)
val sc = ServiceContainer(grafanaPort, s"http://localhost:$grafanaPort", name)
f.map(_ => sc)
}
private def prometheusConfig = {
val config = IOUtils.resourceToString("/prometheus.yml", UTF_8)
val pattern = "'user-events:9095'"
require(config.contains(pattern), s"Did not found expected pattern $pattern in prometheus config $config")
val targets = s"'$hostIp:$userEventPort', '$hostIp:$owPort'"
config.replace(pattern, targets)
}
private def unzipGrafanaConfig(configDir: File, promUrl: String): Unit = {
val is = getClass.getResourceAsStream("/grafana-config.zip")
if (is != null) {
Unzip(is, configDir)
val configFile = new File(configDir, "provisioning/datasources/datasource.yml")
val config = FileUtils.readFileToString(configFile, UTF_8)
val updatedConfig = config.replace("http://prometheus:9090", promUrl)
FileUtils.write(configFile, updatedConfig, UTF_8)
} else {
logging.warn(
this,
"Did not found the grafana-config.zip in classpath. Make sure its packaged and present in classpath")
}
}
private def newDir(baseDir: File, name: String) = {
val dir = new File(baseDir, name)
FileUtils.forceMkdir(dir)
dir
}
}
| {
"content_hash": "f76c602e2100671f1ab7157f7279b89c",
"timestamp": "",
"source": "github",
"line_count": 144,
"max_line_length": 119,
"avg_line_length": 42.18055555555556,
"alnum_prop": 0.6799473164306882,
"repo_name": "openwhisk/openwhisk",
"id": "359a36feaaac787c1986ad13f9a4c12492aed393",
"size": "6875",
"binary": false,
"copies": "2",
"ref": "refs/heads/master",
"path": "core/standalone/src/main/scala/org/apache/openwhisk/standalone/UserEventLauncher.scala",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "C",
"bytes": "623"
},
{
"name": "Java",
"bytes": "39450"
},
{
"name": "JavaScript",
"bytes": "123430"
},
{
"name": "Python",
"bytes": "35043"
},
{
"name": "Scala",
"bytes": "2026708"
},
{
"name": "Shell",
"bytes": "8596"
},
{
"name": "Swift",
"bytes": "23004"
}
],
"symlink_target": ""
} |
/**
* Module dependencies.
*/
var Transport = require('../transport');
var parser = require('engine.io-parser');
var parseqs = require('parseqs');
var inherit = require('component-inherit');
var yeast = require('yeast');
var debug = require('debug')('engine.io-client:websocket');
var BrowserWebSocket = global.WebSocket || global.MozWebSocket;
/**
* Get either the `WebSocket` or `MozWebSocket` globals
* in the browser or the WebSocket-compatible interface
* exposed by `ws` for Node environment.
*/
var WebSocket = BrowserWebSocket || (typeof window !== 'undefined' ? null : require('ws'));
/**
* Module exports.
*/
module.exports = WS;
/**
* WebSocket transport constructor.
*
* @api {Object} connection options
* @api public
*/
function WS(opts){
var forceBase64 = (opts && opts.forceBase64);
if (forceBase64) {
this.supportsBinary = false;
}
this.perMessageDeflate = opts.perMessageDeflate;
Transport.call(this, opts);
}
/**
* Inherits from Transport.
*/
inherit(WS, Transport);
/**
* Transport name.
*
* @api public
*/
WS.prototype.name = 'websocket';
/*
* WebSockets support binary
*/
WS.prototype.supportsBinary = true;
/**
* Opens socket.
*
* @api private
*/
WS.prototype.doOpen = function(){
if (!this.check()) {
// let probe timeout
return;
}
var self = this;
var uri = this.uri();
var protocols = void(0);
var opts = {
agent: this.agent,
perMessageDeflate: this.perMessageDeflate
};
// SSL options for Node.js client
opts.pfx = this.pfx;
opts.key = this.key;
opts.passphrase = this.passphrase;
opts.cert = this.cert;
opts.ca = this.ca;
opts.ciphers = this.ciphers;
opts.rejectUnauthorized = this.rejectUnauthorized;
if (this.extraHeaders) {
opts.headers = this.extraHeaders;
}
this.ws = BrowserWebSocket ? new WebSocket(uri) : new WebSocket(uri, protocols, opts);
if (this.ws.binaryType === undefined) {
this.supportsBinary = false;
}
if (this.ws.supports && this.ws.supports.binary) {
this.supportsBinary = true;
this.ws.binaryType = 'buffer';
} else {
this.ws.binaryType = 'arraybuffer';
}
this.addEventListeners();
};
/**
* Adds event listeners to the socket
*
* @api private
*/
WS.prototype.addEventListeners = function(){
var self = this;
this.ws.onopen = function(){
self.onOpen();
};
this.ws.onclose = function(){
self.onClose();
};
this.ws.onmessage = function(ev){
self.onData(ev.data);
};
this.ws.onerror = function(e){
self.onError('websocket error', e);
};
};
/**
* Override `onData` to use a timer on iOS.
* See: https://gist.github.com/mloughran/2052006
*
* @api private
*/
if ('undefined' != typeof navigator
&& /iPad|iPhone|iPod/i.test(navigator.userAgent)) {
WS.prototype.onData = function(data){
var self = this;
setTimeout(function(){
Transport.prototype.onData.call(self, data);
}, 0);
};
}
/**
* Writes data to socket.
*
* @param {Array} array of packets.
* @api private
*/
WS.prototype.write = function(packets){
var self = this;
this.writable = false;
// encodePacket efficient as it uses WS framing
// no need for encodePayload
var total = packets.length;
for (var i = 0, l = total; i < l; i++) {
(function(packet) {
parser.encodePacket(packet, self.supportsBinary, function(data) {
if (!BrowserWebSocket) {
// always create a new object (GH-437)
var opts = {};
if (packet.options) {
opts.compress = packet.options.compress;
}
if (self.perMessageDeflate) {
var len = 'string' == typeof data ? global.Buffer.byteLength(data) : data.length;
if (len < self.perMessageDeflate.threshold) {
opts.compress = false;
}
}
}
//Sometimes the websocket has already been closed but the browser didn't
//have a chance of informing us about it yet, in that case send will
//throw an error
try {
if (BrowserWebSocket) {
// TypeError is thrown when passing the second argument on Safari
self.ws.send(data);
} else {
self.ws.send(data, opts);
}
} catch (e){
debug('websocket closed before onclose event');
}
--total || done();
});
})(packets[i]);
}
function done(){
self.emit('flush');
// fake drain
// defer to next tick to allow Socket to clear writeBuffer
setTimeout(function(){
self.writable = true;
self.emit('drain');
}, 0);
}
};
/**
* Called upon close
*
* @api private
*/
WS.prototype.onClose = function(){
Transport.prototype.onClose.call(this);
};
/**
* Closes socket.
*
* @api private
*/
WS.prototype.doClose = function(){
if (typeof this.ws !== 'undefined') {
this.ws.close();
}
};
/**
* Generates uri for connection.
*
* @api private
*/
WS.prototype.uri = function(){
var query = this.query || {};
var schema = this.secure ? 'wss' : 'ws';
var port = '';
// avoid port if default for schema
if (this.port && (('wss' == schema && this.port != 443)
|| ('ws' == schema && this.port != 80))) {
port = ':' + this.port;
}
// append timestamp to URI
if (this.timestampRequests) {
query[this.timestampParam] = yeast();
}
// communicate binary support capabilities
if (!this.supportsBinary) {
query.b64 = 1;
}
query = parseqs.encode(query);
// prepend ? to query
if (query.length) {
query = '?' + query;
}
var ipv6 = this.hostname.indexOf(':') !== -1;
return schema + '://' + (ipv6 ? '[' + this.hostname + ']' : this.hostname) + port + this.path + query;
};
/**
* Feature detection for WebSocket.
*
* @return {Boolean} whether this transport is available.
* @api public
*/
WS.prototype.check = function(){
return !!WebSocket && !('__initialize' in WebSocket && this.name === WS.prototype.name);
};
| {
"content_hash": "d2a1392894008fcac585c8cfb43aeaf7",
"timestamp": "",
"source": "github",
"line_count": 283,
"max_line_length": 104,
"avg_line_length": 22.15547703180212,
"alnum_prop": 0.5824561403508772,
"repo_name": "CoderHam/WebScraper",
"id": "71affd8b5715f14d51d91d35ce9af2e2f39b3460",
"size": "6270",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "node_modules/node-phantom/node_modules/socket.io/node_modules/socket.io-client/node_modules/engine.io-client/lib/transports/websocket.js",
"mode": "33261",
"license": "apache-2.0",
"language": [
{
"name": "HTML",
"bytes": "1070"
},
{
"name": "JavaScript",
"bytes": "6347"
}
],
"symlink_target": ""
} |
<?php
namespace Zendvn\Validator;
use Zend\Validator\AbstractValidator;
class CheckCourseID extends AbstractValidator{
const INVALID = 'checkCourseIDInvalid';
protected $messageTemplates = array(
self::INVALID => "Id không đúng quy định",
);
protected $messageVariables = array(
'pattern' => array('options' => 'pattern'),
);
protected $options = array(
'pattern' => 0,
);
public function __construct($options = array())
{
if (!is_array($options)) {
$options = func_get_args();
$temp['pattern'] = array_shift($options);
$options = $temp;
}
parent::__construct($options);
}
public function getPattern()
{
return $this->options['pattern'];
}
public function setPattern($pattern)
{
$this->options['pattern'] = $pattern;
return $this;
}
public function isValid($value) {
$pattern = $this->getPattern();
if (preg_match('#'.$pattern.'#', $value) == false) {
$this->error(self::INVALID);
return false;
}
return true;
}
} | {
"content_hash": "ab00f4ad81b7dc57caa5f76191601075",
"timestamp": "",
"source": "github",
"line_count": 55,
"max_line_length": 54,
"avg_line_length": 19.436363636363637,
"alnum_prop": 0.5968194574368568,
"repo_name": "caohoangnam/ecommerce",
"id": "b8aeb1eb93fb6b3775b19dd01cf45b810159195e",
"size": "1075",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "library/Zendvn/Validator/CheckCourseID.php",
"mode": "33188",
"license": "bsd-3-clause",
"language": [
{
"name": "ApacheConf",
"bytes": "1465"
},
{
"name": "Batchfile",
"bytes": "203"
},
{
"name": "CSS",
"bytes": "1854671"
},
{
"name": "CoffeeScript",
"bytes": "6903"
},
{
"name": "Go",
"bytes": "6808"
},
{
"name": "HTML",
"bytes": "1525582"
},
{
"name": "JavaScript",
"bytes": "6052969"
},
{
"name": "Makefile",
"bytes": "3195"
},
{
"name": "PHP",
"bytes": "252833"
},
{
"name": "Python",
"bytes": "5596"
},
{
"name": "Ruby",
"bytes": "1266"
},
{
"name": "Shell",
"bytes": "1345"
}
],
"symlink_target": ""
} |
package com.vcredit.utils;
import android.app.Activity;
import android.content.Context;
import android.content.Intent;
import android.net.Uri;
import android.os.Environment;
import android.os.StatFs;
import com.vcredit.service.DownloadService;
/**
* Created by wangzhengji on 2016/3/9.
*/
public class DownloadUtils {
/**
* 判断存储空间大小是否满足条件
*
* @param sizeByte
* @return
*/
public static boolean isAvaiableSpace(float sizeByte) {
boolean ishasSpace = false;
if (Environment.getExternalStorageState().equals(
Environment.MEDIA_MOUNTED)) {
String sdcard = Environment.getExternalStorageDirectory().getPath();
StatFs statFs = new StatFs(sdcard);
long blockSize = statFs.getBlockSize();
long blocks = statFs.getAvailableBlocks();
float availableSpare = blocks * blockSize;
if (availableSpare > (sizeByte + 1024 * 1024)) {
ishasSpace = true;
}
}
return ishasSpace;
}
/**
* 开始安装apk文件
*
* @param context
* @param localFilePath
*/
public static void installApkByGuide(Context context, String localFilePath) {
Intent intent = new Intent(Intent.ACTION_VIEW);
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
intent.setDataAndType(Uri.parse("file://" + localFilePath),
"application/vnd.android.package-archive");
context.startActivity(intent);
}
/**
* 下载
*
* @param url 下载链接
* @param context
* @param fileName 文件名
* @param size 文件大小(b)
*/
public static void startDownload(String url, Context context,
String fileName, Float size) {
if (CommonUtils.detectSdcardIsExist()) {
if (isAvaiableSpace(size)) {
Intent intent = new Intent(context, DownloadService.class);
intent.putExtra("downloadUrl", url);
intent.putExtra("fileName", fileName);
context.startService(intent);
} else {
TooltipUtils.showToastS((Activity) context, "存储卡空间不足");
}
} else {
TooltipUtils.showToastS((Activity) context, "请检查存储卡是否安装");
}
}
}
| {
"content_hash": "310c4c83f473cb52016e73dbdfb36274",
"timestamp": "",
"source": "github",
"line_count": 76,
"max_line_length": 81,
"avg_line_length": 30.5,
"alnum_prop": 0.5901639344262295,
"repo_name": "shibenli/app-android",
"id": "6fe0e5e95c1e6118624a036a40bd8f08b77b7503",
"size": "2422",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "app/src/main/java/com/vcredit/utils/DownloadUtils.java",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "IDL",
"bytes": "556"
},
{
"name": "Java",
"bytes": "298011"
},
{
"name": "Shell",
"bytes": "109"
}
],
"symlink_target": ""
} |
package seava.ad.domain.impl.system;
import java.io.Serializable;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.PrePersist;
import javax.persistence.PreUpdate;
import javax.persistence.Table;
import org.hibernate.validator.constraints.NotBlank;
import seava.j4e.domain.impl.AbstractAuditable;
/** Define rules to dynamically linked objects. */
@Entity
@Table(name = TargetRule.TABLE_NAME)
public class TargetRule extends AbstractAuditable implements Serializable {
public static final String TABLE_NAME = "AD_TARGET_RULE";
private static final long serialVersionUID = -8865917134914502125L;
@NotBlank
@Column(name = "SOURCEREFID", nullable = false, length = 64)
private String sourceRefId;
@NotBlank
@Column(name = "TARGETALIAS", nullable = false, length = 255)
private String targetAlias;
@NotBlank
@Column(name = "TARGETTYPE", nullable = false, length = 255)
private String targetType;
public String getSourceRefId() {
return this.sourceRefId;
}
public void setSourceRefId(String sourceRefId) {
this.sourceRefId = sourceRefId;
}
public String getTargetAlias() {
return this.targetAlias;
}
public void setTargetAlias(String targetAlias) {
this.targetAlias = targetAlias;
}
public String getTargetType() {
return this.targetType;
}
public void setTargetType(String targetType) {
this.targetType = targetType;
}
@PrePersist
public void prePersist() {
super.prePersist();
}
@PreUpdate
public void preUpdate() {
super.preUpdate();
}
}
| {
"content_hash": "e4bdc7e59749b0e503b4980264e563cf",
"timestamp": "",
"source": "github",
"line_count": 68,
"max_line_length": 75,
"avg_line_length": 22.63235294117647,
"alnum_prop": 0.7615334632878492,
"repo_name": "seava/seava.mod.ad",
"id": "e91d14c666504f1dd642b83b79991b775051dc35",
"size": "1712",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "seava.mod.ad.domain/src/main/java/seava/ad/domain/impl/system/TargetRule.java",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "Java",
"bytes": "511817"
},
{
"name": "JavaScript",
"bytes": "46698"
},
{
"name": "Shell",
"bytes": "396"
}
],
"symlink_target": ""
} |
ACCEPTED
#### According to
World Register of Marine Species
#### Published in
Notes Lab. Paleont. Univ. Geneve 8 (1-5): 45.
#### Original name
null
### Remarks
null | {
"content_hash": "b0a4661f6abd06c1565689761e765bbc",
"timestamp": "",
"source": "github",
"line_count": 13,
"max_line_length": 45,
"avg_line_length": 12.923076923076923,
"alnum_prop": 0.6845238095238095,
"repo_name": "mdoering/backbone",
"id": "af1a7bd7d436c5cdcb29cb4de5600a12fb9f5ef4",
"size": "219",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "life/Chromista/Globotextulariidae/Rhumblerella/README.md",
"mode": "33188",
"license": "apache-2.0",
"language": [],
"symlink_target": ""
} |
using Slagen.Domain.Services;
namespace Slagen.Domain.Specs.Stubs
{
public class TestCommandHandler : ICommandHandler<TestCommand>
{
public TestCommand CommandHandled { get; private set; }
public void Handle(IUserSession userIssuingCommand, TestCommand command)
{
CommandHandled = command;
if (NotifyObservers != null) NotifyObservers(new TestEvent(command));
}
public event DomainEvent NotifyObservers;
}
} | {
"content_hash": "6273fab45d3dcd73792ff1497b3b63c7",
"timestamp": "",
"source": "github",
"line_count": 17,
"max_line_length": 81,
"avg_line_length": 28.647058823529413,
"alnum_prop": 0.6817248459958932,
"repo_name": "AcklenAvenue/slagen",
"id": "cb5615eee0c625b0bad60bebbe84de59c45544a4",
"size": "489",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "src/Slagen.Domain.Specs/Stubs/TestCommandHandler.cs",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "C#",
"bytes": "114865"
},
{
"name": "JavaScript",
"bytes": "8274"
},
{
"name": "Ruby",
"bytes": "3087"
},
{
"name": "Shell",
"bytes": "35"
}
],
"symlink_target": ""
} |
package main
import (
"context"
"encoding/json"
"errors"
"github.com/aws/aws-sdk-go-v2/aws"
"io/ioutil"
"testing"
"time"
"github.com/aws/aws-sdk-go-v2/service/cloudwatchlogs"
)
type CWLGetLogEventsImpl struct{}
func (dt CWLGetLogEventsImpl) GetLogEvents(ctx context.Context,
params *cloudwatchlogs.GetLogEventsInput,
optFns ...func(*cloudwatchlogs.Options)) (*cloudwatchlogs.GetLogEventsOutput, error) {
return &cloudwatchlogs.GetLogEventsOutput{}, nil
}
type Config struct {
LogGroup string `json:"LogGroup"`
LogStream string `json:"LogStream"`
}
var configFileName = "config.json"
var globalConfig Config
func populateConfiguration(t *testing.T) error {
content, err := ioutil.ReadFile(configFileName)
if err != nil {
return err
}
text := string(content)
err = json.Unmarshal([]byte(text), &globalConfig)
if err != nil {
return err
}
if globalConfig.LogGroup == "" || globalConfig.LogStream == "" {
msg := "You must supply a value for LogGroup and LogStream in " + configFileName
return errors.New(msg)
}
return nil
}
func TestGetLogEvents(t *testing.T) {
thisTime := time.Now()
nowString := thisTime.Format("2006-01-02 15:04:05 Monday")
t.Log("Starting unit test at " + nowString)
err := populateConfiguration(t)
if err != nil {
t.Fatal(err)
}
input := &cloudwatchlogs.GetLogEventsInput{
LogGroupName: aws.String(globalConfig.LogGroup),
LogStreamName: aws.String(globalConfig.LogStream),
}
api := &CWLGetLogEventsImpl{}
_, err = GetEvents(context.Background(), *api, input)
if err != nil {
t.Log("Got an error ...:")
t.Log(err)
return
}
t.Log("Fetched Log Events successfully")
}
| {
"content_hash": "ae88f64724eac050dcace1a88c56e519",
"timestamp": "",
"source": "github",
"line_count": 78,
"max_line_length": 87,
"avg_line_length": 21.26923076923077,
"alnum_prop": 0.7094635322483424,
"repo_name": "awsdocs/aws-doc-sdk-examples",
"id": "dc91e0db2a8a5f8d77f719bf5d6438c3f043217f",
"size": "1659",
"binary": false,
"copies": "1",
"ref": "refs/heads/main",
"path": "gov2/cloudwatch/GetLogEvents/GetLogEventsv2_test.go",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "ABAP",
"bytes": "476653"
},
{
"name": "Batchfile",
"bytes": "900"
},
{
"name": "C",
"bytes": "3852"
},
{
"name": "C#",
"bytes": "2051923"
},
{
"name": "C++",
"bytes": "943634"
},
{
"name": "CMake",
"bytes": "82068"
},
{
"name": "CSS",
"bytes": "33378"
},
{
"name": "Dockerfile",
"bytes": "2243"
},
{
"name": "Go",
"bytes": "1764292"
},
{
"name": "HTML",
"bytes": "319090"
},
{
"name": "Java",
"bytes": "4966853"
},
{
"name": "JavaScript",
"bytes": "1655476"
},
{
"name": "Jupyter Notebook",
"bytes": "9749"
},
{
"name": "Kotlin",
"bytes": "1099902"
},
{
"name": "Makefile",
"bytes": "4922"
},
{
"name": "PHP",
"bytes": "1220594"
},
{
"name": "Python",
"bytes": "2507509"
},
{
"name": "Ruby",
"bytes": "500331"
},
{
"name": "Rust",
"bytes": "558811"
},
{
"name": "Shell",
"bytes": "63776"
},
{
"name": "Swift",
"bytes": "267325"
},
{
"name": "TypeScript",
"bytes": "119632"
}
],
"symlink_target": ""
} |
package glue
import (
. "github.com/smartystreets/goconvey/convey"
"testing"
)
func TestGlue(t *testing.T) {
Convey("Accessing the built glue", t, func() {
Convey("File 'gluetest' should equal \"Hello world!\\n\"", func() {
So(GetGlue("gluetest"), ShouldEqual, "Hello world!\n")
})
})
}
| {
"content_hash": "4902dcf263f8dfc13bfcfeea035e89a4",
"timestamp": "",
"source": "github",
"line_count": 14,
"max_line_length": 69,
"avg_line_length": 21.428571428571427,
"alnum_prop": 0.6533333333333333,
"repo_name": "carbonsrv/carbon",
"id": "18e11372062b2477eaa5b5f3212610748bc06b8c",
"size": "300",
"binary": false,
"copies": "2",
"ref": "refs/heads/master",
"path": "modules/glue/glue_test.go",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "Go",
"bytes": "72374"
},
{
"name": "HTML",
"bytes": "44"
},
{
"name": "Lua",
"bytes": "62857"
},
{
"name": "Makefile",
"bytes": "903"
},
{
"name": "Shell",
"bytes": "158"
}
],
"symlink_target": ""
} |
using System.Collections.Generic;
namespace Azure.Management.Resources.Models
{
/// <summary> Resource information. </summary>
public partial class GenericResourceAutoGenerated : Resource
{
/// <summary> Initializes a new instance of GenericResourceAutoGenerated. </summary>
public GenericResourceAutoGenerated()
{
}
/// <summary> Initializes a new instance of GenericResourceAutoGenerated. </summary>
/// <param name="id"> Resource ID. </param>
/// <param name="name"> Resource name. </param>
/// <param name="type"> Resource type. </param>
/// <param name="location"> Resource location. </param>
/// <param name="tags"> Resource tags. </param>
/// <param name="managedBy"> ID of the resource that manages this resource. </param>
/// <param name="sku"> The SKU of the resource. </param>
/// <param name="identity"> The identity of the resource. </param>
internal GenericResourceAutoGenerated(string id, string name, string type, string location, IDictionary<string, string> tags, string managedBy, SkuAutoGenerated sku, IdentityAutoGenerated2 identity) : base(id, name, type, location, tags)
{
ManagedBy = managedBy;
Sku = sku;
Identity = identity;
}
/// <summary> ID of the resource that manages this resource. </summary>
public string ManagedBy { get; set; }
/// <summary> The SKU of the resource. </summary>
public SkuAutoGenerated Sku { get; set; }
/// <summary> The identity of the resource. </summary>
public IdentityAutoGenerated2 Identity { get; set; }
}
}
| {
"content_hash": "e667ce422767a61475b33ebcdec396e6",
"timestamp": "",
"source": "github",
"line_count": 36,
"max_line_length": 245,
"avg_line_length": 47.138888888888886,
"alnum_prop": 0.6352386564525634,
"repo_name": "hyonholee/azure-sdk-for-net",
"id": "5e07ab4fef157a5c5db03502b5c1348c64241e8f",
"size": "1835",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "sdk/resources/Azure.Management.Resources/src/Generated/Models/GenericResourceAutoGenerated.cs",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "Batchfile",
"bytes": "16774"
},
{
"name": "C#",
"bytes": "37415276"
},
{
"name": "HTML",
"bytes": "234899"
},
{
"name": "JavaScript",
"bytes": "7875"
},
{
"name": "PowerShell",
"bytes": "273940"
},
{
"name": "Shell",
"bytes": "13061"
},
{
"name": "Smarty",
"bytes": "11135"
},
{
"name": "TypeScript",
"bytes": "143209"
}
],
"symlink_target": ""
} |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.