text
stringlengths 2
1.04M
| meta
dict |
---|---|
package io.quarkus.kubernetes.deployment;
import io.dekorate.kubernetes.decorator.Decorator;
import io.dekorate.kubernetes.decorator.NamedResourceDecorator;
import io.dekorate.kubernetes.decorator.ResourceProvidingDecorator;
import io.fabric8.kubernetes.api.model.ObjectMeta;
import io.fabric8.kubernetes.api.model.ServiceSpecFluent;
/**
* A decorator for applying a serviceType to the container
*/
public class ApplyServiceTypeDecorator extends NamedResourceDecorator<ServiceSpecFluent> {
private final String type;
public ApplyServiceTypeDecorator(String name, String type) {
super(name);
this.type = type;
}
@Override
public void andThenVisit(ServiceSpecFluent service, ObjectMeta resourceMeta) {
service.withType(type);
}
@Override
public Class<? extends Decorator>[] after() {
return new Class[] { ResourceProvidingDecorator.class };
}
}
| {
"content_hash": "dfbcba2f169b58469c836da5afb185da",
"timestamp": "",
"source": "github",
"line_count": 31,
"max_line_length": 90,
"avg_line_length": 29.677419354838708,
"alnum_prop": 0.7543478260869565,
"repo_name": "quarkusio/quarkus",
"id": "9fc2d3e5ccb730f72621b7778ab3eca5e4df9c04",
"size": "920",
"binary": false,
"copies": "1",
"ref": "refs/heads/main",
"path": "extensions/kubernetes/vanilla/deployment/src/main/java/io/quarkus/kubernetes/deployment/ApplyServiceTypeDecorator.java",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "ANTLR",
"bytes": "23342"
},
{
"name": "Batchfile",
"bytes": "13096"
},
{
"name": "CSS",
"bytes": "6685"
},
{
"name": "Dockerfile",
"bytes": "459"
},
{
"name": "FreeMarker",
"bytes": "8106"
},
{
"name": "Groovy",
"bytes": "16133"
},
{
"name": "HTML",
"bytes": "1418749"
},
{
"name": "Java",
"bytes": "38584810"
},
{
"name": "JavaScript",
"bytes": "90960"
},
{
"name": "Kotlin",
"bytes": "704351"
},
{
"name": "Mustache",
"bytes": "13191"
},
{
"name": "Scala",
"bytes": "9756"
},
{
"name": "Shell",
"bytes": "71729"
}
],
"symlink_target": ""
} |
namespace base {
#if defined(OS_POSIX)
namespace internal {
// This class is friended by ThreadLocalStorage.
class ThreadLocalStorageTestInternal {
public:
static bool HasBeenDestroyed() {
return ThreadLocalStorage::HasBeenDestroyed();
}
};
} // namespace internal
#endif // defined(OS_POSIX)
namespace {
const int kInitialTlsValue = 0x5555;
const int kFinalTlsValue = 0x7777;
// How many times must a destructor be called before we really are done.
const int kNumberDestructorCallRepetitions = 3;
void ThreadLocalStorageCleanup(void* value);
ThreadLocalStorage::Slot& TLSSlot() {
static NoDestructor<ThreadLocalStorage::Slot> slot(
&ThreadLocalStorageCleanup);
return *slot;
}
class ThreadLocalStorageRunner : public DelegateSimpleThread::Delegate {
public:
explicit ThreadLocalStorageRunner(int* tls_value_ptr)
: tls_value_ptr_(tls_value_ptr) {}
ThreadLocalStorageRunner(const ThreadLocalStorageRunner&) = delete;
ThreadLocalStorageRunner& operator=(const ThreadLocalStorageRunner&) = delete;
~ThreadLocalStorageRunner() override = default;
void Run() override {
*tls_value_ptr_ = kInitialTlsValue;
TLSSlot().Set(tls_value_ptr_);
int* ptr = static_cast<int*>(TLSSlot().Get());
EXPECT_EQ(ptr, tls_value_ptr_);
EXPECT_EQ(*ptr, kInitialTlsValue);
*tls_value_ptr_ = 0;
ptr = static_cast<int*>(TLSSlot().Get());
EXPECT_EQ(ptr, tls_value_ptr_);
EXPECT_EQ(*ptr, 0);
*ptr = kFinalTlsValue + kNumberDestructorCallRepetitions;
}
private:
raw_ptr<int> tls_value_ptr_;
};
void ThreadLocalStorageCleanup(void *value) {
int *ptr = reinterpret_cast<int*>(value);
// Destructors should never be called with a NULL.
ASSERT_NE(reinterpret_cast<int*>(NULL), ptr);
if (*ptr == kFinalTlsValue)
return; // We've been called enough times.
ASSERT_LT(kFinalTlsValue, *ptr);
ASSERT_GE(kFinalTlsValue + kNumberDestructorCallRepetitions, *ptr);
--*ptr; // Move closer to our target.
// Tell tls that we're not done with this thread, and still need destruction.
TLSSlot().Set(value);
}
#if defined(OS_POSIX)
constexpr intptr_t kDummyValue = 0xABCD;
constexpr size_t kKeyCount = 20;
// The order in which pthread keys are destructed is not specified by the POSIX
// specification. Hopefully, of the 20 keys we create, some of them should be
// destroyed after the TLS key is destroyed.
class UseTLSDuringDestructionRunner {
public:
UseTLSDuringDestructionRunner() = default;
UseTLSDuringDestructionRunner(const UseTLSDuringDestructionRunner&) = delete;
UseTLSDuringDestructionRunner& operator=(
const UseTLSDuringDestructionRunner&) = delete;
// The order in which pthread_key destructors are called is not well defined.
// Hopefully, by creating 10 both before and after initializing TLS on the
// thread, at least 1 will be called after TLS destruction.
void Run() {
ASSERT_FALSE(internal::ThreadLocalStorageTestInternal::HasBeenDestroyed());
// Create 10 pthread keys before initializing TLS on the thread.
size_t slot_index = 0;
for (; slot_index < 10; ++slot_index) {
CreateTlsKeyWithDestructor(slot_index);
}
// Initialize the Chrome TLS system. It's possible that base::Thread has
// already initialized Chrome TLS, but we don't rely on that.
slot_.Set(reinterpret_cast<void*>(kDummyValue));
// Create 10 pthread keys after initializing TLS on the thread.
for (; slot_index < kKeyCount; ++slot_index) {
CreateTlsKeyWithDestructor(slot_index);
}
}
bool teardown_works_correctly() { return teardown_works_correctly_; }
private:
struct TLSState {
pthread_key_t key;
raw_ptr<bool> teardown_works_correctly;
};
// The POSIX TLS destruction API takes as input a single C-function, which is
// called with the current |value| of a (key, value) pair. We need this
// function to do two things: set the |value| to nullptr, which requires
// knowing the associated |key|, and update the |teardown_works_correctly_|
// state.
//
// To accomplish this, we set the value to an instance of TLSState, which
// contains |key| as well as a pointer to |teardown_works_correctly|.
static void ThreadLocalDestructor(void* value) {
TLSState* state = static_cast<TLSState*>(value);
int result = pthread_setspecific(state->key, nullptr);
ASSERT_EQ(result, 0);
// If this path is hit, then the thread local destructor was called after
// the Chrome-TLS destructor and the internal state was updated correctly.
// No further checks are necessary.
if (internal::ThreadLocalStorageTestInternal::HasBeenDestroyed()) {
*(state->teardown_works_correctly) = true;
return;
}
// If this path is hit, then the thread local destructor was called before
// the Chrome-TLS destructor is hit. The ThreadLocalStorage::Slot should
// still function correctly.
ASSERT_EQ(reinterpret_cast<intptr_t>(slot_.Get()), kDummyValue);
}
void CreateTlsKeyWithDestructor(size_t index) {
ASSERT_LT(index, kKeyCount);
tls_states_[index].teardown_works_correctly = &teardown_works_correctly_;
int result = pthread_key_create(
&(tls_states_[index].key),
UseTLSDuringDestructionRunner::ThreadLocalDestructor);
ASSERT_EQ(result, 0);
result = pthread_setspecific(tls_states_[index].key, &tls_states_[index]);
ASSERT_EQ(result, 0);
}
static base::ThreadLocalStorage::Slot slot_;
bool teardown_works_correctly_ = false;
TLSState tls_states_[kKeyCount];
};
base::ThreadLocalStorage::Slot UseTLSDuringDestructionRunner::slot_;
void* UseTLSTestThreadRun(void* input) {
UseTLSDuringDestructionRunner* runner =
static_cast<UseTLSDuringDestructionRunner*>(input);
runner->Run();
return nullptr;
}
#endif // defined(OS_POSIX)
} // namespace
TEST(ThreadLocalStorageTest, Basics) {
ThreadLocalStorage::Slot slot;
slot.Set(reinterpret_cast<void*>(123));
int value = reinterpret_cast<intptr_t>(slot.Get());
EXPECT_EQ(value, 123);
}
#if defined(THREAD_SANITIZER)
// Do not run the test under ThreadSanitizer. Because this test iterates its
// own TSD destructor for the maximum possible number of times, TSan can't jump
// in after the last destructor invocation, therefore the destructor remains
// unsynchronized with the following users of the same TSD slot. This results
// in race reports between the destructor and functions in other tests.
#define MAYBE_TLSDestructors DISABLED_TLSDestructors
#else
#define MAYBE_TLSDestructors TLSDestructors
#endif
TEST(ThreadLocalStorageTest, MAYBE_TLSDestructors) {
// Create a TLS index with a destructor. Create a set of
// threads that set the TLS, while the destructor cleans it up.
// After the threads finish, verify that the value is cleaned up.
const int kNumThreads = 5;
int values[kNumThreads];
ThreadLocalStorageRunner* thread_delegates[kNumThreads];
DelegateSimpleThread* threads[kNumThreads];
// Spawn the threads.
for (int index = 0; index < kNumThreads; index++) {
values[index] = kInitialTlsValue;
thread_delegates[index] = new ThreadLocalStorageRunner(&values[index]);
threads[index] = new DelegateSimpleThread(thread_delegates[index],
"tls thread");
threads[index]->Start();
}
// Wait for the threads to finish.
for (int index = 0; index < kNumThreads; index++) {
threads[index]->Join();
delete threads[index];
delete thread_delegates[index];
// Verify that the destructor was called and that we reset.
EXPECT_EQ(values[index], kFinalTlsValue);
}
}
TEST(ThreadLocalStorageTest, TLSReclaim) {
// Creates and destroys many TLS slots and ensures they all zero-inited.
for (int i = 0; i < 1000; ++i) {
ThreadLocalStorage::Slot slot(nullptr);
EXPECT_EQ(nullptr, slot.Get());
slot.Set(reinterpret_cast<void*>(0xBAADF00D));
EXPECT_EQ(reinterpret_cast<void*>(0xBAADF00D), slot.Get());
}
}
#if defined(OS_POSIX)
// Unlike POSIX, Windows does not iterate through the OS TLS to cleanup any
// values there. Instead a per-module thread destruction function is called.
// However, it is not possible to perform a check after this point (as the code
// is detached from the thread), so this check remains POSIX only.
TEST(ThreadLocalStorageTest, UseTLSDuringDestruction) {
UseTLSDuringDestructionRunner runner;
pthread_t thread;
int result = pthread_create(&thread, nullptr, UseTLSTestThreadRun, &runner);
ASSERT_EQ(result, 0);
result = pthread_join(thread, nullptr);
ASSERT_EQ(result, 0);
EXPECT_TRUE(runner.teardown_works_correctly());
}
#endif // defined(OS_POSIX)
} // namespace base
| {
"content_hash": "95bd8a54959b4040de2850399ebbf93f",
"timestamp": "",
"source": "github",
"line_count": 255,
"max_line_length": 80,
"avg_line_length": 34.082352941176474,
"alnum_prop": 0.7190196755264067,
"repo_name": "ric2b/Vivaldi-browser",
"id": "9a3266919d6d70d519d6f6e2ef9c0af2d67f3564",
"size": "9338",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "chromium/base/threading/thread_local_storage_unittest.cc",
"mode": "33188",
"license": "bsd-3-clause",
"language": [],
"symlink_target": ""
} |
namespace std _GLIBCXX_VISIBILITY(default)
{
_GLIBCXX_BEGIN_NAMESPACE_VERSION
// Definitions for locale::id of standard facets that are specialized.
locale::id codecvt<char, char, mbstate_t>::id;
#ifdef _GLIBCXX_USE_WCHAR_T
locale::id codecvt<wchar_t, char, mbstate_t>::id;
#endif
codecvt<char, char, mbstate_t>::
codecvt(size_t __refs)
: __codecvt_abstract_base<char, char, mbstate_t>(__refs),
_M_c_locale_codecvt(_S_get_c_locale())
{ }
codecvt<char, char, mbstate_t>::
codecvt(__c_locale __cloc, size_t __refs)
: __codecvt_abstract_base<char, char, mbstate_t>(__refs),
_M_c_locale_codecvt(_S_clone_c_locale(__cloc))
{ }
codecvt<char, char, mbstate_t>::
~codecvt()
{ _S_destroy_c_locale(_M_c_locale_codecvt); }
codecvt_base::result
codecvt<char, char, mbstate_t>::
do_out(state_type&, const intern_type* __from,
const intern_type*, const intern_type*& __from_next,
extern_type* __to, extern_type*,
extern_type*& __to_next) const
{
// _GLIBCXX_RESOLVE_LIB_DEFECTS
// According to the resolution of DR19, "If returns noconv [...]
// there are no changes to the values in [to, to_limit)."
__from_next = __from;
__to_next = __to;
return noconv;
}
codecvt_base::result
codecvt<char, char, mbstate_t>::
do_unshift(state_type&, extern_type* __to,
extern_type*, extern_type*& __to_next) const
{
__to_next = __to;
return noconv;
}
codecvt_base::result
codecvt<char, char, mbstate_t>::
do_in(state_type&, const extern_type* __from,
const extern_type*, const extern_type*& __from_next,
intern_type* __to, intern_type*, intern_type*& __to_next) const
{
// _GLIBCXX_RESOLVE_LIB_DEFECTS
// According to the resolution of DR19, "If returns noconv [...]
// there are no changes to the values in [to, to_limit)."
__from_next = __from;
__to_next = __to;
return noconv;
}
int
codecvt<char, char, mbstate_t>::
do_encoding() const throw()
{ return 1; }
bool
codecvt<char, char, mbstate_t>::
do_always_noconv() const throw()
{ return true; }
int
codecvt<char, char, mbstate_t>::
do_length (state_type&, const extern_type* __from,
const extern_type* __end, size_t __max) const
{
size_t __d = static_cast<size_t>(__end - __from);
return std::min(__max, __d);
}
int
codecvt<char, char, mbstate_t>::
do_max_length() const throw()
{ return 1; }
#ifdef _GLIBCXX_USE_WCHAR_T
// codecvt<wchar_t, char, mbstate_t> required specialization
codecvt<wchar_t, char, mbstate_t>::
codecvt(size_t __refs)
: __codecvt_abstract_base<wchar_t, char, mbstate_t>(__refs),
_M_c_locale_codecvt(_S_get_c_locale())
{ }
codecvt<wchar_t, char, mbstate_t>::
codecvt(__c_locale __cloc, size_t __refs)
: __codecvt_abstract_base<wchar_t, char, mbstate_t>(__refs),
_M_c_locale_codecvt(_S_clone_c_locale(__cloc))
{ }
codecvt<wchar_t, char, mbstate_t>::
~codecvt()
{ _S_destroy_c_locale(_M_c_locale_codecvt); }
codecvt_base::result
codecvt<wchar_t, char, mbstate_t>::
do_unshift(state_type&, extern_type* __to,
extern_type*, extern_type*& __to_next) const
{
// XXX Probably wrong for stateful encodings
__to_next = __to;
return noconv;
}
bool
codecvt<wchar_t, char, mbstate_t>::
do_always_noconv() const throw()
{ return false; }
#endif // _GLIBCXX_USE_WCHAR_T
_GLIBCXX_END_NAMESPACE_VERSION
} // namespace
| {
"content_hash": "5a5f79fd72c5cd907409171943326342",
"timestamp": "",
"source": "github",
"line_count": 125,
"max_line_length": 72,
"avg_line_length": 27.696,
"alnum_prop": 0.6221837088388215,
"repo_name": "the-linix-project/linix-kernel-source",
"id": "fdb0896caa29d7d3bbbf8bf83f1396a8dee7f1f8",
"size": "4589",
"binary": false,
"copies": "35",
"ref": "refs/heads/master",
"path": "gccsrc/gcc-4.7.2/libstdc++-v3/src/c++98/codecvt.cc",
"mode": "33188",
"license": "bsd-2-clause",
"language": [
{
"name": "Ada",
"bytes": "38139979"
},
{
"name": "Assembly",
"bytes": "3723477"
},
{
"name": "Awk",
"bytes": "83739"
},
{
"name": "C",
"bytes": "103607293"
},
{
"name": "C#",
"bytes": "55726"
},
{
"name": "C++",
"bytes": "38577421"
},
{
"name": "CLIPS",
"bytes": "6933"
},
{
"name": "CSS",
"bytes": "32588"
},
{
"name": "Emacs Lisp",
"bytes": "13451"
},
{
"name": "FORTRAN",
"bytes": "4294984"
},
{
"name": "GAP",
"bytes": "13089"
},
{
"name": "Go",
"bytes": "11277335"
},
{
"name": "Haskell",
"bytes": "2415"
},
{
"name": "Java",
"bytes": "45298678"
},
{
"name": "JavaScript",
"bytes": "6265"
},
{
"name": "Matlab",
"bytes": "56"
},
{
"name": "OCaml",
"bytes": "148372"
},
{
"name": "Objective-C",
"bytes": "995127"
},
{
"name": "Objective-C++",
"bytes": "436045"
},
{
"name": "PHP",
"bytes": "12361"
},
{
"name": "Pascal",
"bytes": "40318"
},
{
"name": "Perl",
"bytes": "358808"
},
{
"name": "Python",
"bytes": "60178"
},
{
"name": "SAS",
"bytes": "1711"
},
{
"name": "Scilab",
"bytes": "258457"
},
{
"name": "Shell",
"bytes": "2610907"
},
{
"name": "Tcl",
"bytes": "17983"
},
{
"name": "TeX",
"bytes": "1455571"
},
{
"name": "XSLT",
"bytes": "156419"
}
],
"symlink_target": ""
} |
/*
* This file is part of Cleanflight, Betaflight and INAV.
*
* Cleanflight and Betaflight are free software. You can redistribute
* this software and/or modify this software under the terms of the
* GNU General Public License as published by the Free Software
* Foundation, either version 3 of the License, or (at your option)
* any later version.
*
* Cleanflight, Betaflight and INAV are distributed in the hope that they
* will be useful, but WITHOUT ANY WARRANTY; without even the implied
* warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
* See the GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this software.
*
* If not, see <http://www.gnu.org/licenses/>.
*/
#define MSP2_BETAFLIGHT_BIND 0x3000
#define MSP2_MOTOR_OUTPUT_REORDERING 0x3001
#define MSP2_SET_MOTOR_OUTPUT_REORDERING 0x3002
| {
"content_hash": "36a38b26d83d9f415a00a19e5d388fc7",
"timestamp": "",
"source": "github",
"line_count": 23,
"max_line_length": 73,
"avg_line_length": 40.69565217391305,
"alnum_prop": 0.7393162393162394,
"repo_name": "rtlopez/esp-fc",
"id": "ba07b5b7475b73de29c5793fb4adaccede742a7d",
"size": "936",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "lib/betaflight/src/msp/msp_protocol_v2_betaflight.h",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "C",
"bytes": "202571"
},
{
"name": "C++",
"bytes": "505796"
},
{
"name": "Shell",
"bytes": "221"
}
],
"symlink_target": ""
} |
<!DOCTYPE html>
<html>
<head>
<link href="css/awsdocs.css" rel="stylesheet" type="text/css">
<script type="text/javascript" src="js/jquery.min.js"></script>
<script type="text/javascript" src="js/awsdocs.min.js"></script>
<meta charset="utf-8">
</head>
<body>
<div id="content" style="padding: 10px 30px;">
<h1 class="topictitle" id="aws-properties-opsworks-stack-chefconfiguration">AWS OpsWorks ChefConfiguration Type</h1><p>Describes the Chef configuration for the <a href="aws-resource-opsworks-stack.html">AWS::OpsWorks::Stack</a> resource type. For more information, see
<a href="https://docs.aws.amazon.com/opsworks/latest/APIReference/API_ChefConfiguration.html">ChefConfiguration</a> in
the <em>AWS OpsWorks Stacks API Reference</em>.
</p><h2 id="w2ab1c21c10d183c38c17b5">Syntax</h2><div id="JSON" name="JSON" class="section langfilter">
<h3 id="aws-properties-opsworks-stack-chefconfiguration-syntax.json">JSON</h3>
<pre class="programlisting"><div class="code-btn-container"><div class="btn-copy-code" title="Copy"></div><div class="btn-dark-theme" title="Dark theme" title-dark="Dark theme" title-light="Light theme"></div></div><code class="nohighlight">{
"<a href="aws-properties-opsworks-stack-chefconfiguration.html#cfn-opsworks-chefconfiguration-berkshelfversion">BerkshelfVersion</a>" : <em class="replaceable"><code>String</code></em>,
"<a href="aws-properties-opsworks-stack-chefconfiguration.html#cfn-opsworks-chefconfiguration-manageberkshelf">ManageBerkshelf</a>" : <em class="replaceable"><code>Boolean</code></em>
}</code></pre>
</div><div id="YAML" name="YAML" class="section langfilter">
<h3 id="aws-properties-opsworks-stack-chefconfiguration-syntax.yaml">YAML</h3>
<pre class="programlisting"><div class="code-btn-container"><div class="btn-copy-code" title="Copy"></div><div class="btn-dark-theme" title="Dark theme" title-dark="Dark theme" title-light="Light theme"></div></div><code class="nohighlight"><a href="aws-properties-opsworks-stack-chefconfiguration.html#cfn-opsworks-chefconfiguration-berkshelfversion">BerkshelfVersion</a>: <em class="replaceable"><code>String</code></em>
<a href="aws-properties-opsworks-stack-chefconfiguration.html#cfn-opsworks-chefconfiguration-manageberkshelf">ManageBerkshelf</a>: <em class="replaceable"><code>Boolean</code></em>
</code></pre>
</div><h2 id="w2ab1c21c10d183c38c17b7">Properties</h2><div class="variablelist">
<dl>
<dt><a id="cfn-opsworks-chefconfiguration-berkshelfversion"></a><span class="term"><code class="literal">BerkshelfVersion</code></span></dt>
<dd>
<p>The Berkshelf version.</p>
<p><em>Required</em>: No
</p>
<p><em>Type</em>: String
</p>
</dd>
<dt><a id="cfn-opsworks-chefconfiguration-manageberkshelf"></a><span class="term"><code class="literal">ManageBerkshelf</code></span></dt>
<dd>
<p>Whether to enable Berkshelf.</p>
<p><em>Required</em>: No
</p>
<p><em>Type</em>: Boolean
</p>
</dd>
</dl>
</div></div>
</body>
</html> | {
"content_hash": "3c5c59fc2229ab3d9d6d36dba24f377e",
"timestamp": "",
"source": "github",
"line_count": 65,
"max_line_length": 446,
"avg_line_length": 65.43076923076923,
"alnum_prop": 0.5116388431695273,
"repo_name": "pdhodgkinson/AWSCloudFormationTemplateReference-dash-docset",
"id": "ba40b7dbfa578208d7a3209a2bef3538730d6858",
"size": "4253",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "AWS_CloudFormation_Template_Reference.docset/Contents/Resources/Documents/aws-properties-opsworks-stack-chefconfiguration.html",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "CSS",
"bytes": "63666"
},
{
"name": "HTML",
"bytes": "13504124"
},
{
"name": "JavaScript",
"bytes": "9850"
}
],
"symlink_target": ""
} |
import tornado.web
import tornado.websocket
import tornado.ioloop
# This is our WebSocketHandler - it handles the messages
# from the tornado server
class WebSocketHandler(tornado.websocket.WebSocketHandler):
# the client connected
def open(self):
print "New client connected"
self.write_message("You are connected")
# the client sent the message
def on_message(self, message):
self.write_message(message)
# client disconnected
def on_close(self):
print "Client disconnected"
# start a new WebSocket Application
# use "/" as the root, and the
# WebSocketHandler as our handler
application = tornado.web.Application([
(r"/ws", WebSocketHandler),
])
# start the tornado server on port 8888
if __name__ == "__main__":
application.listen(8888)
tornado.ioloop.IOLoop.instance().start()
| {
"content_hash": "792059954dfe4cfe35ca7f83ef3789fc",
"timestamp": "",
"source": "github",
"line_count": 31,
"max_line_length": 59,
"avg_line_length": 26.322580645161292,
"alnum_prop": 0.7377450980392157,
"repo_name": "juliansotoca/smartevents",
"id": "140e0dbe14a41757bc4c183eb6be9a78656ae7ab",
"size": "857",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "echoServer.py",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "CSS",
"bytes": "5999"
},
{
"name": "HTML",
"bytes": "22872"
},
{
"name": "JavaScript",
"bytes": "17217"
},
{
"name": "Python",
"bytes": "18467"
}
],
"symlink_target": ""
} |
namespace Yfer.ZedGraph.Extension.Test
{
partial class OscViewForm
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.IContainer components = null;
/// <summary>
/// Clean up any resources being used.
/// </summary>
/// <param name="disposing">true if managed resources should be disposed; otherwise, false.</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.components = new System.ComponentModel.Container();
this.zedGraphControl1 = new global::ZedGraph.ZedGraphControl();
this.button1 = new System.Windows.Forms.Button();
this.button2 = new System.Windows.Forms.Button();
this.button3 = new System.Windows.Forms.Button();
this.checkBox1 = new System.Windows.Forms.CheckBox();
this.SuspendLayout();
//
// zedGraphControl1
//
this.zedGraphControl1.Anchor = ((System.Windows.Forms.AnchorStyles)((((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Bottom)
| System.Windows.Forms.AnchorStyles.Left)
| System.Windows.Forms.AnchorStyles.Right)));
this.zedGraphControl1.IsAntiAlias = true;
this.zedGraphControl1.Location = new System.Drawing.Point(12, 12);
this.zedGraphControl1.Name = "zedGraphControl1";
this.zedGraphControl1.ScrollGrace = 0;
this.zedGraphControl1.ScrollMaxX = 0;
this.zedGraphControl1.ScrollMaxY = 0;
this.zedGraphControl1.ScrollMaxY2 = 0;
this.zedGraphControl1.ScrollMinX = 0;
this.zedGraphControl1.ScrollMinY = 0;
this.zedGraphControl1.ScrollMinY2 = 0;
this.zedGraphControl1.Size = new System.Drawing.Size(616, 280);
this.zedGraphControl1.TabIndex = 0;
//
// button1
//
this.button1.Anchor = ((System.Windows.Forms.AnchorStyles)((System.Windows.Forms.AnchorStyles.Bottom | System.Windows.Forms.AnchorStyles.Right)));
this.button1.Location = new System.Drawing.Point(471, 298);
this.button1.Name = "button1";
this.button1.Size = new System.Drawing.Size(76, 28);
this.button1.TabIndex = 1;
this.button1.Text = "Pass";
this.button1.UseVisualStyleBackColor = true;
this.button1.Click += new System.EventHandler(this.button1_Click);
//
// button2
//
this.button2.Anchor = ((System.Windows.Forms.AnchorStyles)((System.Windows.Forms.AnchorStyles.Bottom | System.Windows.Forms.AnchorStyles.Right)));
this.button2.Location = new System.Drawing.Point(553, 298);
this.button2.Name = "button2";
this.button2.Size = new System.Drawing.Size(76, 28);
this.button2.TabIndex = 2;
this.button2.Text = "Fail";
this.button2.UseVisualStyleBackColor = true;
this.button2.Click += new System.EventHandler(this.button2_Click);
//
// button3
//
this.button3.Anchor = ((System.Windows.Forms.AnchorStyles)((System.Windows.Forms.AnchorStyles.Bottom | System.Windows.Forms.AnchorStyles.Left)));
this.button3.Location = new System.Drawing.Point(12, 298);
this.button3.Name = "button3";
this.button3.Size = new System.Drawing.Size(80, 28);
this.button3.TabIndex = 3;
this.button3.Text = "Random";
this.button3.UseVisualStyleBackColor = true;
this.button3.Click += new System.EventHandler(this.button3_Click);
//
// checkBox1
//
this.checkBox1.AutoSize = true;
this.checkBox1.Location = new System.Drawing.Point(99, 298);
this.checkBox1.Name = "checkBox1";
this.checkBox1.Size = new System.Drawing.Size(44, 17);
this.checkBox1.TabIndex = 4;
this.checkBox1.Text = "Log";
this.checkBox1.UseVisualStyleBackColor = true;
this.checkBox1.CheckedChanged += new System.EventHandler(this.checkBox1_CheckedChanged);
//
// OscViewForm
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(641, 332);
this.Controls.Add(this.checkBox1);
this.Controls.Add(this.button3);
this.Controls.Add(this.button2);
this.Controls.Add(this.button1);
this.Controls.Add(this.zedGraphControl1);
this.Name = "OscViewForm";
this.StartPosition = System.Windows.Forms.FormStartPosition.CenterScreen;
this.Text = "OscViewForm";
this.TopMost = true;
this.Load += new System.EventHandler(this.OscViewForm_Load);
this.ResumeLayout(false);
this.PerformLayout();
}
#endregion
private global::ZedGraph.ZedGraphControl zedGraphControl1;
private System.Windows.Forms.Button button1;
private System.Windows.Forms.Button button2;
private System.Windows.Forms.Button button3;
private System.Windows.Forms.CheckBox checkBox1;
}
} | {
"content_hash": "c092943e41d62d53e8db092fa8962ee3",
"timestamp": "",
"source": "github",
"line_count": 129,
"max_line_length": 164,
"avg_line_length": 46.031007751937985,
"alnum_prop": 0.5966655439541934,
"repo_name": "yfer/ZedGraph.Extension",
"id": "db0158f79b1643b9d6cea396d43e2a9a49f096a1",
"size": "5940",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "Yfer.ZedGraph.Extension.Test/OscViewForm.Designer.cs",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "C#",
"bytes": "17837"
}
],
"symlink_target": ""
} |
package configuration
import (
"github.com/cloudfoundry/cli/cf/configuration/core_config"
"github.com/cloudfoundry/cli/cf/models"
)
func NewRepository() core_config.Repository {
return core_config.NewRepositoryFromPersistor(NewFakePersistor(), func(err error) {
panic(err)
})
}
func NewRepositoryWithAccessToken(tokenInfo core_config.TokenInfo) core_config.Repository {
accessToken, err := EncodeAccessToken(tokenInfo)
if err != nil {
panic(err)
}
config := NewRepository()
config.SetAccessToken(accessToken)
return config
}
func NewRepositoryWithDefaults() core_config.Repository {
configRepo := NewRepositoryWithAccessToken(core_config.TokenInfo{
UserGuid: "my-user-guid",
Username: "my-user",
})
configRepo.SetSpaceFields(models.SpaceFields{
Name: "my-space",
Guid: "my-space-guid",
})
configRepo.SetOrganizationFields(models.OrganizationFields{
Name: "my-org",
Guid: "my-org-guid",
})
return configRepo
}
| {
"content_hash": "442c79056544983ad04b06748ea68b1b",
"timestamp": "",
"source": "github",
"line_count": 41,
"max_line_length": 91,
"avg_line_length": 23.146341463414632,
"alnum_prop": 0.7565858798735511,
"repo_name": "AmitRoushan/cli",
"id": "f5f92f2a6109bf7511c0800dddbfa02b7ae240f5",
"size": "949",
"binary": false,
"copies": "5",
"ref": "refs/heads/master",
"path": "testhelpers/configuration/test_config.go",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "Go",
"bytes": "4516238"
},
{
"name": "HTML",
"bytes": "1728"
},
{
"name": "Makefile",
"bytes": "1930"
},
{
"name": "PowerShell",
"bytes": "682"
},
{
"name": "Ruby",
"bytes": "5867"
},
{
"name": "Shell",
"bytes": "18063"
}
],
"symlink_target": ""
} |
export { TropicalWarning20 as default } from "../../";
| {
"content_hash": "7e05d3f84a5208ad50c596f99776f2d1",
"timestamp": "",
"source": "github",
"line_count": 1,
"max_line_length": 54,
"avg_line_length": 55,
"alnum_prop": 0.6545454545454545,
"repo_name": "georgemarshall/DefinitelyTyped",
"id": "72fa8bb4b4929fcbb8f12548612f031ab9362baa",
"size": "55",
"binary": false,
"copies": "24",
"ref": "refs/heads/master",
"path": "types/carbon__icons-react/es/tropical-warning/20.d.ts",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "JavaScript",
"bytes": "16338312"
},
{
"name": "Ruby",
"bytes": "40"
},
{
"name": "Shell",
"bytes": "73"
},
{
"name": "TypeScript",
"bytes": "17728346"
}
],
"symlink_target": ""
} |
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/xhtml;charset=UTF-8"/>
<meta http-equiv="X-UA-Compatible" content="IE=9"/>
<meta name="generator" content="Doxygen 1.8.9.1"/>
<title>V8 API Reference Guide for io.js v1.8.3 - v1.8.4: v8::SealHandleScope Class Reference</title>
<link href="tabs.css" rel="stylesheet" type="text/css"/>
<script type="text/javascript" src="jquery.js"></script>
<script type="text/javascript" src="dynsections.js"></script>
<link href="search/search.css" rel="stylesheet" type="text/css"/>
<script type="text/javascript" src="search/searchdata.js"></script>
<script type="text/javascript" src="search/search.js"></script>
<script type="text/javascript">
$(document).ready(function() { init_search(); });
</script>
<link href="doxygen.css" rel="stylesheet" type="text/css" />
</head>
<body>
<div id="top"><!-- do not remove this div, it is closed by doxygen! -->
<div id="titlearea">
<table cellspacing="0" cellpadding="0">
<tbody>
<tr style="height: 56px;">
<td style="padding-left: 0.5em;">
<div id="projectname">V8 API Reference Guide for io.js v1.8.3 - v1.8.4
</div>
</td>
</tr>
</tbody>
</table>
</div>
<!-- end header part -->
<!-- Generated by Doxygen 1.8.9.1 -->
<script type="text/javascript">
var searchBox = new SearchBox("searchBox", "search",false,'Search');
</script>
<div id="navrow1" class="tabs">
<ul class="tablist">
<li><a href="index.html"><span>Main Page</span></a></li>
<li><a href="namespaces.html"><span>Namespaces</span></a></li>
<li class="current"><a href="annotated.html"><span>Classes</span></a></li>
<li><a href="files.html"><span>Files</span></a></li>
<li><a href="examples.html"><span>Examples</span></a></li>
<li>
<div id="MSearchBox" class="MSearchBoxInactive">
<span class="left">
<img id="MSearchSelect" src="search/mag_sel.png"
onmouseover="return searchBox.OnSearchSelectShow()"
onmouseout="return searchBox.OnSearchSelectHide()"
alt=""/>
<input type="text" id="MSearchField" value="Search" accesskey="S"
onfocus="searchBox.OnSearchFieldFocus(true)"
onblur="searchBox.OnSearchFieldFocus(false)"
onkeyup="searchBox.OnSearchFieldChange(event)"/>
</span><span class="right">
<a id="MSearchClose" href="javascript:searchBox.CloseResultsWindow()"><img id="MSearchCloseImg" border="0" src="search/close.png" alt=""/></a>
</span>
</div>
</li>
</ul>
</div>
<div id="navrow2" class="tabs2">
<ul class="tablist">
<li><a href="annotated.html"><span>Class List</span></a></li>
<li><a href="classes.html"><span>Class Index</span></a></li>
<li><a href="hierarchy.html"><span>Class Hierarchy</span></a></li>
<li><a href="functions.html"><span>Class Members</span></a></li>
</ul>
</div>
<!-- window showing the filter options -->
<div id="MSearchSelectWindow"
onmouseover="return searchBox.OnSearchSelectShow()"
onmouseout="return searchBox.OnSearchSelectHide()"
onkeydown="return searchBox.OnSearchSelectKey(event)">
</div>
<!-- iframe showing the search results (closed by default) -->
<div id="MSearchResultsWindow">
<iframe src="javascript:void(0)" frameborder="0"
name="MSearchResults" id="MSearchResults">
</iframe>
</div>
<div id="nav-path" class="navpath">
<ul>
<li class="navelem"><a class="el" href="namespacev8.html">v8</a></li><li class="navelem"><a class="el" href="classv8_1_1_seal_handle_scope.html">SealHandleScope</a></li> </ul>
</div>
</div><!-- top -->
<div class="header">
<div class="summary">
<a href="#pub-methods">Public Member Functions</a> |
<a href="classv8_1_1_seal_handle_scope-members.html">List of all members</a> </div>
<div class="headertitle">
<div class="title">v8::SealHandleScope Class Reference</div> </div>
</div><!--header-->
<div class="contents">
<table class="memberdecls">
<tr class="heading"><td colspan="2"><h2 class="groupheader"><a name="pub-methods"></a>
Public Member Functions</h2></td></tr>
<tr class="memitem:acfdab75cc53b53d3ba1a50ab5f4fe16e"><td class="memItemLeft" align="right" valign="top"><a class="anchor" id="acfdab75cc53b53d3ba1a50ab5f4fe16e"></a>
 </td><td class="memItemRight" valign="bottom"><b>SealHandleScope</b> (<a class="el" href="classv8_1_1_isolate.html">Isolate</a> *isolate)</td></tr>
<tr class="separator:acfdab75cc53b53d3ba1a50ab5f4fe16e"><td class="memSeparator" colspan="2"> </td></tr>
</table>
<hr/>The documentation for this class was generated from the following file:<ul>
<li>deps/v8/include/<a class="el" href="v8_8h_source.html">v8.h</a></li>
</ul>
</div><!-- contents -->
<!-- start footer part -->
<hr class="footer"/><address class="footer"><small>
Generated on Tue Aug 11 2015 23:49:46 for V8 API Reference Guide for io.js v1.8.3 - v1.8.4 by  <a href="http://www.doxygen.org/index.html">
<img class="footer" src="doxygen.png" alt="doxygen"/>
</a> 1.8.9.1
</small></address>
</body>
</html>
| {
"content_hash": "e7f9a9852d60f8df0dd9484a2e3a109f",
"timestamp": "",
"source": "github",
"line_count": 116,
"max_line_length": 176,
"avg_line_length": 45.26724137931034,
"alnum_prop": 0.654351552085317,
"repo_name": "v8-dox/v8-dox.github.io",
"id": "c5b7b1f6ee5b4ee864d79bef4f539c78794a94eb",
"size": "5251",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "78de5f8/html/classv8_1_1_seal_handle_scope.html",
"mode": "33188",
"license": "mit",
"language": [],
"symlink_target": ""
} |
mip-yuezhua-video {
max-height: 100%;
overflow: auto;
box-sizing: border-box;
}
mip-yuezhua-video a {
color: #fff;
}
mip-yuezhua-video .mip-yuezhua-video-countdown {
position: absolute;
top: 5px;
right: 5px;
}
#MIP-LLIGTBOX-MASK {
position: fixed !important;
top: 0 !important;
left: 0 !important;
width: 100% !important;
height: 100% !important;
background-image: none !important;
background: rgba(0, 0, 0, 0.8);
z-index: 10000 !important;
display: none;
}
| {
"content_hash": "4884156222419033e21834154e78cd65",
"timestamp": "",
"source": "github",
"line_count": 24,
"max_line_length": 48,
"avg_line_length": 20.416666666666668,
"alnum_prop": 0.6673469387755102,
"repo_name": "mipengine/mip-extensions-platform",
"id": "3edb39fb4d0422f17a0353e9408ed5b999eb439c",
"size": "490",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "mip-yuezhua-video/mip-yuezhua-video.css",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "CSS",
"bytes": "966670"
},
{
"name": "HTML",
"bytes": "24624"
},
{
"name": "JavaScript",
"bytes": "11914398"
}
],
"symlink_target": ""
} |
package org.antlr.v4.codegen.model;
import org.antlr.v4.Tool;
import org.antlr.v4.codegen.OutputModelFactory;
import org.antlr.v4.tool.Grammar;
import org.antlr.v4.tool.ast.ActionAST;
import java.util.HashMap;
import java.util.Map;
public abstract class OutputFile extends OutputModelObject {
public final String fileName;
public final String grammarFileName;
public final String ANTLRVersion;
public final String TokenLabelType;
public final String InputSymbolType;
public OutputFile(OutputModelFactory factory, String fileName) {
super(factory);
this.fileName = fileName;
Grammar g = factory.getGrammar();
grammarFileName = g.fileName;
ANTLRVersion = Tool.VERSION;
TokenLabelType = g.getOptionString("TokenLabelType");
InputSymbolType = TokenLabelType;
}
public Map<String, Action> buildNamedActions(Grammar g) {
Map<String, Action> namedActions = new HashMap<String, Action>();
for (String name : g.namedActions.keySet()) {
ActionAST ast = g.namedActions.get(name);
namedActions.put(name, new Action(factory, ast));
}
return namedActions;
}
}
| {
"content_hash": "b18532f8ba98e3d2bf2b1ea089152e07",
"timestamp": "",
"source": "github",
"line_count": 38,
"max_line_length": 68,
"avg_line_length": 29.789473684210527,
"alnum_prop": 0.7385159010600707,
"repo_name": "chandler14362/antlr4",
"id": "e238dae1b6d54c91687bbacfe107e2243fb89eac",
"size": "1331",
"binary": false,
"copies": "4",
"ref": "refs/heads/master",
"path": "tool/src/org/antlr/v4/codegen/model/OutputFile.java",
"mode": "33188",
"license": "bsd-3-clause",
"language": [
{
"name": "ANTLR",
"bytes": "168002"
},
{
"name": "Batchfile",
"bytes": "3188"
},
{
"name": "C",
"bytes": "9558"
},
{
"name": "C#",
"bytes": "1120270"
},
{
"name": "C++",
"bytes": "981935"
},
{
"name": "CMake",
"bytes": "18345"
},
{
"name": "GAP",
"bytes": "110858"
},
{
"name": "Go",
"bytes": "366101"
},
{
"name": "Java",
"bytes": "2732641"
},
{
"name": "JavaScript",
"bytes": "421267"
},
{
"name": "Makefile",
"bytes": "1513"
},
{
"name": "Objective-C",
"bytes": "408"
},
{
"name": "Objective-C++",
"bytes": "27915"
},
{
"name": "PowerShell",
"bytes": "6138"
},
{
"name": "Python",
"bytes": "1352058"
},
{
"name": "Shell",
"bytes": "9122"
},
{
"name": "Swift",
"bytes": "949079"
}
],
"symlink_target": ""
} |
package com.benstatertots.brewerydb.di;
/**
* From Android's architecture samples:
* https://github.com/googlesamples/android-architecture-components/blob/master/GithubBrowserSample/app/src/main/java/com/android/example/github/di/ViewModelKey.java
*/
import android.arch.lifecycle.ViewModel;
import java.lang.annotation.Documented;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
import dagger.MapKey;
@Documented
@Target({ElementType.METHOD})
@Retention(RetentionPolicy.RUNTIME)
@MapKey
@interface ViewModelKey {
Class<? extends ViewModel> value();
}
| {
"content_hash": "4a7c1f2c131b65c6c960c9682a5bc508",
"timestamp": "",
"source": "github",
"line_count": 24,
"max_line_length": 165,
"avg_line_length": 28.208333333333332,
"alnum_prop": 0.8064992614475628,
"repo_name": "stupergenius/BreweryDB-Android",
"id": "fc6088b7851c99d0553a4bf29fe80cca5273d9ba",
"size": "677",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "app/src/main/java/com/benstatertots/brewerydb/di/ViewModelKey.java",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "Java",
"bytes": "35724"
}
],
"symlink_target": ""
} |
#include "signverifymessagedialog.h"
#include "ui_signverifymessagedialog.h"
#include "addressbookpage.h"
#include "base58.h"
#include "guiutil.h"
#include "init.h"
#include "main.h"
#include "optionsmodel.h"
#include "walletmodel.h"
#include "wallet.h"
#include <QClipboard>
#include <string>
#include <vector>
SignVerifyMessageDialog::SignVerifyMessageDialog(QWidget *parent) :
QDialog(parent),
ui(new Ui::SignVerifyMessageDialog),
model(0)
{
ui->setupUi(this);
#if (QT_VERSION >= 0x040700)
/* Do not move this to the XML file, Qt before 4.7 will choke on it */
ui->addressIn_SM->setPlaceholderText(tr("Enter a HongKongCoin address (e.g. 1NS17iag9jJgTHD1VXjvLCEnZuQ3rJDE9L)"));
ui->signatureOut_SM->setPlaceholderText(tr("Click \"Sign Message\" to generate signature"));
ui->addressIn_VM->setPlaceholderText(tr("Enter a HongKongCoin address (e.g. 1NS17iag9jJgTHD1VXjvLCEnZuQ3rJDE9L)"));
ui->signatureIn_VM->setPlaceholderText(tr("Enter HongKongCoin signature"));
#endif
GUIUtil::setupAddressWidget(ui->addressIn_SM, this);
GUIUtil::setupAddressWidget(ui->addressIn_VM, this);
ui->addressIn_SM->installEventFilter(this);
ui->messageIn_SM->installEventFilter(this);
ui->signatureOut_SM->installEventFilter(this);
ui->addressIn_VM->installEventFilter(this);
ui->messageIn_VM->installEventFilter(this);
ui->signatureIn_VM->installEventFilter(this);
ui->signatureOut_SM->setFont(GUIUtil::hongkongcoinAddressFont());
ui->signatureIn_VM->setFont(GUIUtil::hongkongcoinAddressFont());
}
SignVerifyMessageDialog::~SignVerifyMessageDialog()
{
delete ui;
}
void SignVerifyMessageDialog::setModel(WalletModel *model)
{
this->model = model;
}
void SignVerifyMessageDialog::setAddress_SM(const QString &address)
{
ui->addressIn_SM->setText(address);
ui->messageIn_SM->setFocus();
}
void SignVerifyMessageDialog::setAddress_VM(const QString &address)
{
ui->addressIn_VM->setText(address);
ui->messageIn_VM->setFocus();
}
void SignVerifyMessageDialog::showTab_SM(bool fShow)
{
ui->tabWidget->setCurrentIndex(0);
if (fShow)
this->show();
}
void SignVerifyMessageDialog::showTab_VM(bool fShow)
{
ui->tabWidget->setCurrentIndex(1);
if (fShow)
this->show();
}
void SignVerifyMessageDialog::on_addressBookButton_SM_clicked()
{
if (model && model->getAddressTableModel())
{
AddressBookPage dlg(AddressBookPage::ForSending, AddressBookPage::ReceivingTab, this);
dlg.setModel(model->getAddressTableModel());
if (dlg.exec())
{
setAddress_SM(dlg.getReturnValue());
}
}
}
void SignVerifyMessageDialog::on_pasteButton_SM_clicked()
{
setAddress_SM(QApplication::clipboard()->text());
}
void SignVerifyMessageDialog::on_signMessageButton_SM_clicked()
{
/* Clear old signature to ensure users don't get confused on error with an old signature displayed */
ui->signatureOut_SM->clear();
CHongKongCoinAddress addr(ui->addressIn_SM->text().toStdString());
if (!addr.IsValid())
{
ui->addressIn_SM->setValid(false);
ui->statusLabel_SM->setStyleSheet("QLabel { color: red; }");
ui->statusLabel_SM->setText(tr("The entered address is invalid.") + QString(" ") + tr("Please check the address and try again."));
return;
}
CKeyID keyID;
if (!addr.GetKeyID(keyID))
{
ui->addressIn_SM->setValid(false);
ui->statusLabel_SM->setStyleSheet("QLabel { color: red; }");
ui->statusLabel_SM->setText(tr("The entered address does not refer to a key.") + QString(" ") + tr("Please check the address and try again."));
return;
}
WalletModel::UnlockContext ctx(model->requestUnlock());
if (!ctx.isValid())
{
ui->statusLabel_SM->setStyleSheet("QLabel { color: red; }");
ui->statusLabel_SM->setText(tr("Wallet unlock was cancelled."));
return;
}
CKey key;
if (!pwalletMain->GetKey(keyID, key))
{
ui->statusLabel_SM->setStyleSheet("QLabel { color: red; }");
ui->statusLabel_SM->setText(tr("Private key for the entered address is not available."));
return;
}
CDataStream ss(SER_GETHASH, 0);
ss << strMessageMagic;
ss << ui->messageIn_SM->document()->toPlainText().toStdString();
std::vector<unsigned char> vchSig;
if (!key.SignCompact(Hash(ss.begin(), ss.end()), vchSig))
{
ui->statusLabel_SM->setStyleSheet("QLabel { color: red; }");
ui->statusLabel_SM->setText(QString("<nobr>") + tr("Message signing failed.") + QString("</nobr>"));
return;
}
ui->statusLabel_SM->setStyleSheet("QLabel { color: green; }");
ui->statusLabel_SM->setText(QString("<nobr>") + tr("Message signed.") + QString("</nobr>"));
ui->signatureOut_SM->setText(QString::fromStdString(EncodeBase64(&vchSig[0], vchSig.size())));
}
void SignVerifyMessageDialog::on_copySignatureButton_SM_clicked()
{
QApplication::clipboard()->setText(ui->signatureOut_SM->text());
}
void SignVerifyMessageDialog::on_clearButton_SM_clicked()
{
ui->addressIn_SM->clear();
ui->messageIn_SM->clear();
ui->signatureOut_SM->clear();
ui->statusLabel_SM->clear();
ui->addressIn_SM->setFocus();
}
void SignVerifyMessageDialog::on_addressBookButton_VM_clicked()
{
if (model && model->getAddressTableModel())
{
AddressBookPage dlg(AddressBookPage::ForSending, AddressBookPage::SendingTab, this);
dlg.setModel(model->getAddressTableModel());
if (dlg.exec())
{
setAddress_VM(dlg.getReturnValue());
}
}
}
void SignVerifyMessageDialog::on_verifyMessageButton_VM_clicked()
{
CHongKongCoinAddress addr(ui->addressIn_VM->text().toStdString());
if (!addr.IsValid())
{
ui->addressIn_VM->setValid(false);
ui->statusLabel_VM->setStyleSheet("QLabel { color: red; }");
ui->statusLabel_VM->setText(tr("The entered address is invalid.") + QString(" ") + tr("Please check the address and try again."));
return;
}
CKeyID keyID;
if (!addr.GetKeyID(keyID))
{
ui->addressIn_VM->setValid(false);
ui->statusLabel_VM->setStyleSheet("QLabel { color: red; }");
ui->statusLabel_VM->setText(tr("The entered address does not refer to a key.") + QString(" ") + tr("Please check the address and try again."));
return;
}
bool fInvalid = false;
std::vector<unsigned char> vchSig = DecodeBase64(ui->signatureIn_VM->text().toStdString().c_str(), &fInvalid);
if (fInvalid)
{
ui->signatureIn_VM->setValid(false);
ui->statusLabel_VM->setStyleSheet("QLabel { color: red; }");
ui->statusLabel_VM->setText(tr("The signature could not be decoded.") + QString(" ") + tr("Please check the signature and try again."));
return;
}
CDataStream ss(SER_GETHASH, 0);
ss << strMessageMagic;
ss << ui->messageIn_VM->document()->toPlainText().toStdString();
CKey key;
if (!key.SetCompactSignature(Hash(ss.begin(), ss.end()), vchSig))
{
ui->signatureIn_VM->setValid(false);
ui->statusLabel_VM->setStyleSheet("QLabel { color: red; }");
ui->statusLabel_VM->setText(tr("The signature did not match the message digest.") + QString(" ") + tr("Please check the signature and try again."));
return;
}
if (!(CHongKongCoinAddress(key.GetPubKey().GetID()) == addr))
{
ui->statusLabel_VM->setStyleSheet("QLabel { color: red; }");
ui->statusLabel_VM->setText(QString("<nobr>") + tr("Message verification failed.") + QString("</nobr>"));
return;
}
ui->statusLabel_VM->setStyleSheet("QLabel { color: green; }");
ui->statusLabel_VM->setText(QString("<nobr>") + tr("Message verified.") + QString("</nobr>"));
}
void SignVerifyMessageDialog::on_clearButton_VM_clicked()
{
ui->addressIn_VM->clear();
ui->signatureIn_VM->clear();
ui->messageIn_VM->clear();
ui->statusLabel_VM->clear();
ui->addressIn_VM->setFocus();
}
bool SignVerifyMessageDialog::eventFilter(QObject *object, QEvent *event)
{
if (event->type() == QEvent::MouseButtonPress || event->type() == QEvent::FocusIn)
{
if (ui->tabWidget->currentIndex() == 0)
{
/* Clear status message on focus change */
ui->statusLabel_SM->clear();
/* Select generated signature */
if (object == ui->signatureOut_SM)
{
ui->signatureOut_SM->selectAll();
return true;
}
}
else if (ui->tabWidget->currentIndex() == 1)
{
/* Clear status message on focus change */
ui->statusLabel_VM->clear();
}
}
return QDialog::eventFilter(object, event);
}
| {
"content_hash": "9c9360f02274fc4bace6371ab824969a",
"timestamp": "",
"source": "github",
"line_count": 274,
"max_line_length": 156,
"avg_line_length": 32.237226277372265,
"alnum_prop": 0.6446280991735537,
"repo_name": "digiwhite/hongkongcoin",
"id": "07350fa691ae29665690fd7f24b90c8a3c29f1df",
"size": "8833",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "src/qt/signverifymessagedialog.cpp",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "C",
"bytes": "94741"
},
{
"name": "C++",
"bytes": "2435947"
},
{
"name": "CSS",
"bytes": "1127"
},
{
"name": "IDL",
"bytes": "13918"
},
{
"name": "Objective-C++",
"bytes": "2734"
},
{
"name": "Python",
"bytes": "3818"
},
{
"name": "Shell",
"bytes": "848"
},
{
"name": "TypeScript",
"bytes": "5260834"
}
],
"symlink_target": ""
} |
using System;
class Money
{
static void Main()
{
//read input
int nStudents = int.Parse(Console.ReadLine());
int sSheets = int.Parse(Console.ReadLine());
double pPrice = double.Parse(Console.ReadLine());
int realm = 400;
//logic
double result;
result = ((nStudents * sSheets) /(double)(realm)) * pPrice;
//print result
Console.WriteLine("{0:F3}",result);
}
}
| {
"content_hash": "20538a238f42be2d83a35a49a8a98781",
"timestamp": "",
"source": "github",
"line_count": 22,
"max_line_length": 67,
"avg_line_length": 20.772727272727273,
"alnum_prop": 0.5536105032822757,
"repo_name": "DanielaIvanova/01.CSharp-Fundamentals",
"id": "c518d368bb994972ce7ca6f320b58972e8e7cff9",
"size": "459",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "Exam1@2February2015Evening/1.Money/Money.cs",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "C#",
"bytes": "163688"
},
{
"name": "Smalltalk",
"bytes": "690"
}
],
"symlink_target": ""
} |
class Profiles::ChatNamesController < Profiles::ApplicationController
before_action :chat_name_token, only: [:new]
before_action :chat_name_params, only: [:new, :create, :deny]
def index
@chat_names = current_user.chat_names
end
def new
end
def create
new_chat_name = current_user.chat_names.new(chat_name_params)
if new_chat_name.save
flash[:notice] = "Authorized #{new_chat_name.chat_name}"
else
flash[:alert] = "Could not authorize chat nickname. Try again!"
end
delete_chat_name_token
redirect_to profile_chat_names_path
end
def deny
delete_chat_name_token
flash[:notice] = "Denied authorization of chat nickname #{chat_name_params[:user_name]}."
redirect_to profile_chat_names_path
end
def destroy
@chat_name = chat_names.find(params[:id])
if @chat_name.destroy
flash[:notice] = "Deleted chat nickname: #{@chat_name.chat_name}!"
else
flash[:alert] = "Could not delete chat nickname #{@chat_name.chat_name}."
end
redirect_to profile_chat_names_path, status: 302
end
private
def delete_chat_name_token
chat_name_token.delete
end
def chat_name_params
@chat_name_params ||= chat_name_token.get || render_404
end
def chat_name_token
return render_404 unless params[:token] || render_404
@chat_name_token ||= Gitlab::ChatNameToken.new(params[:token])
end
def chat_names
@chat_names ||= current_user.chat_names
end
end
| {
"content_hash": "84fe81923ae456d46c2e21384a35624e",
"timestamp": "",
"source": "github",
"line_count": 64,
"max_line_length": 93,
"avg_line_length": 23.125,
"alnum_prop": 0.672972972972973,
"repo_name": "dplarson/gitlabhq",
"id": "2353f0840d672e13f1014dd4ef90c036fdd11d66",
"size": "1480",
"binary": false,
"copies": "5",
"ref": "refs/heads/master",
"path": "app/controllers/profiles/chat_names_controller.rb",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "CSS",
"bytes": "536999"
},
{
"name": "Gherkin",
"bytes": "116570"
},
{
"name": "HTML",
"bytes": "1044163"
},
{
"name": "JavaScript",
"bytes": "2193246"
},
{
"name": "Ruby",
"bytes": "11711128"
},
{
"name": "Shell",
"bytes": "27251"
},
{
"name": "Vue",
"bytes": "196242"
}
],
"symlink_target": ""
} |
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Victor
{
public static partial class Make
{
/// <summary>
/// Makes a new eitherton.
/// </summary>
/// <typeparam name="A">Item type.</typeparam>
/// <param name="yes">"Yes" option.</param>
/// <param name="no">"No" option.</param>
/// <returns></returns>
public static either<A> either<A>(A yes, A no) => new either<A>(yes, no);
}
}
| {
"content_hash": "2e8f7739b13ee29677773ec6da5fb041",
"timestamp": "",
"source": "github",
"line_count": 20,
"max_line_length": 81,
"avg_line_length": 26.95,
"alnum_prop": 0.5807050092764379,
"repo_name": "TheBerkin/Victor",
"id": "cf8063e8ad9555b88cdfdbdf3ecc86f7f0b91d04",
"size": "541",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "Victor/Make.Either.cs",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "C#",
"bytes": "121841"
}
],
"symlink_target": ""
} |
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.sql.models;
import com.azure.core.annotation.Fluent;
import com.fasterxml.jackson.annotation.JsonProperty;
/** The PrivateEndpointProperty model. */
@Fluent
public final class PrivateEndpointProperty {
/*
* Resource id of the private endpoint.
*/
@JsonProperty(value = "id")
private String id;
/**
* Get the id property: Resource id of the private endpoint.
*
* @return the id value.
*/
public String id() {
return this.id;
}
/**
* Set the id property: Resource id of the private endpoint.
*
* @param id the id value to set.
* @return the PrivateEndpointProperty object itself.
*/
public PrivateEndpointProperty withId(String id) {
this.id = id;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
}
}
| {
"content_hash": "5d14ccbdf97196ae37a6832f856093c5",
"timestamp": "",
"source": "github",
"line_count": 46,
"max_line_length": 76,
"avg_line_length": 24.782608695652176,
"alnum_prop": 0.6464912280701754,
"repo_name": "Azure/azure-sdk-for-java",
"id": "913fb225acedca500a0e0e250d7061af5165d435",
"size": "1140",
"binary": false,
"copies": "1",
"ref": "refs/heads/main",
"path": "sdk/resourcemanager/azure-resourcemanager-sql/src/main/java/com/azure/resourcemanager/sql/models/PrivateEndpointProperty.java",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "Batchfile",
"bytes": "8762"
},
{
"name": "Bicep",
"bytes": "15055"
},
{
"name": "CSS",
"bytes": "7676"
},
{
"name": "Dockerfile",
"bytes": "2028"
},
{
"name": "Groovy",
"bytes": "3237482"
},
{
"name": "HTML",
"bytes": "42090"
},
{
"name": "Java",
"bytes": "432409546"
},
{
"name": "JavaScript",
"bytes": "36557"
},
{
"name": "Jupyter Notebook",
"bytes": "95868"
},
{
"name": "PowerShell",
"bytes": "737517"
},
{
"name": "Python",
"bytes": "240542"
},
{
"name": "Scala",
"bytes": "1143898"
},
{
"name": "Shell",
"bytes": "18488"
},
{
"name": "XSLT",
"bytes": "755"
}
],
"symlink_target": ""
} |
#region License
#endregion
using System.Text;
using System.Patterns.UI.Forms;
namespace System.Web.UI.WebControls
{
/// <summary>
/// DropDownListEx
/// </summary>
public class DropDownListEx : DropDownList, IFormControl
{
private string _staticTextSeparator = ", ";
private FormFieldViewMode _renderViewMode;
/// <summary>
/// SingleItemRenderMode
/// </summary>
public enum SingleItemRenderMode
{
/// <summary>
/// UseFieldMode
/// </summary>
UseFieldMode = 0,
/// <summary>
/// RenderHidden
/// </summary>
RenderHidden,
/// <summary>
/// RenderStaticWithHidden
/// </summary>
RenderStaticWithHidden
}
public DropDownListEx() { HasHeaderItem = true; }
public bool AutoSelectFirstItem { get; set; }
public bool AutoSelectIfSingleItem { get; set; }
public bool HasHeaderItem { get; set; }
public FormFieldViewMode ViewMode { get; set; }
#region Option-Groups
public static ListItem CreateBeginGroupListItem(string text)
{
var listItem = new ListItem(text);
listItem.Attributes["group"] = "begin";
return listItem;
}
public static ListItem CreateEndGroupListItem()
{
var listItem = new ListItem();
listItem.Attributes["group"] = "end";
return listItem;
}
//[Match("match with ListBox RenderContents")]
protected override void RenderContents(HtmlTextWriter w)
{
ListItemCollection itemHash = Items;
int itemCount = itemHash.Count;
if (itemCount > 0)
{
bool isA = false;
for (int itemKey = 0; itemKey < itemCount; itemKey++)
{
ListItem listItem = itemHash[itemKey];
if (listItem.Enabled)
switch (listItem.Attributes["group"])
{
case "begin":
w.WriteBeginTag("optgroup");
w.WriteAttribute("label", listItem.Text);
w.Write('>');
break;
case "end":
w.WriteEndTag("optgroup");
break;
default:
w.WriteBeginTag("option");
if (listItem.Selected)
{
if (isA)
VerifyMultiSelect();
isA = true;
w.WriteAttribute("selected", "selected");
}
w.WriteAttribute("value", listItem.Value, true);
if (listItem.Attributes.Count > 0)
listItem.Attributes.Render(w);
if (Page != null)
Page.ClientScript.RegisterForEventValidation(UniqueID, listItem.Value);
w.Write('>');
HttpUtility.HtmlEncode(listItem.Text, w);
w.WriteEndTag("option");
w.WriteLine();
break;
}
}
}
}
#endregion
protected override void OnPreRender(EventArgs e)
{
base.OnPreRender(e);
_renderViewMode = ViewMode;
int itemCountIfListHasSingleItem = (HasHeaderItem ? 2 : 1);
int firstItemIndex = itemCountIfListHasSingleItem - 1;
bool setSelectedIndex = ((SelectedIndex == 0) && ((AutoSelectFirstItem) || ((AutoSelectIfSingleItem) && (Items.Count == itemCountIfListHasSingleItem))));
if (setSelectedIndex)
SelectedIndex = firstItemIndex;
SingleItemRenderMode singleItemRenderMode = SingleItemRenderOption;
if ((singleItemRenderMode != SingleItemRenderMode.UseFieldMode) && (_renderViewMode == FormFieldViewMode.Input) && (Items.Count == itemCountIfListHasSingleItem))
switch (singleItemRenderMode)
{
case SingleItemRenderMode.RenderHidden:
_renderViewMode = FormFieldViewMode.Hidden;
break;
case SingleItemRenderMode.RenderStaticWithHidden:
_renderViewMode = FormFieldViewMode.StaticWithHidden;
break;
}
}
protected override void Render(HtmlTextWriter w)
{
switch (_renderViewMode)
{
case FormFieldViewMode.Static:
RenderStaticText(w);
break;
case FormFieldViewMode.StaticWithHidden:
RenderStaticText(w);
RenderHidden(w);
break;
case FormFieldViewMode.Hidden:
RenderHidden(w);
break;
default:
base.Render(w);
break;
}
}
protected void RenderHidden(HtmlTextWriter w)
{
var b = new StringBuilder();
foreach (ListItem item in Items)
if (item.Selected)
b.Append(item.Value + ",");
if (b.Length > 0)
b.Length--;
w.AddAttribute(HtmlTextWriterAttribute.Type, "hidden");
w.AddAttribute(HtmlTextWriterAttribute.Id, ClientID);
w.AddAttribute(HtmlTextWriterAttribute.Name, UniqueID);
w.AddAttribute(HtmlTextWriterAttribute.Value, b.ToString());
w.RenderBeginTag(HtmlTextWriterTag.Input);
w.RenderEndTag();
}
protected virtual void RenderStaticText(HtmlTextWriter w)
{
string staticTextSeparator = StaticTextSeparator;
var b = new StringBuilder();
foreach (ListItem item in Items)
if (item.Selected)
b.Append(HttpUtility.HtmlEncode(item.Text) + staticTextSeparator);
int staticTextSeparatorLength = staticTextSeparator.Length;
if (b.Length > staticTextSeparatorLength)
b.Length -= staticTextSeparatorLength;
w.AddAttribute(HtmlTextWriterAttribute.Class, "static");
w.RenderBeginTag(HtmlTextWriterTag.Span);
w.Write(b.ToString());
w.RenderEndTag();
}
public SingleItemRenderMode SingleItemRenderOption { get; set; }
public string StaticTextSeparator
{
get { return _staticTextSeparator; }
set
{
if (value == null)
throw new ArgumentNullException("value");
_staticTextSeparator = value;
}
}
}
}
| {
"content_hash": "784d570140daa69e235b5b13377ed69c",
"timestamp": "",
"source": "github",
"line_count": 194,
"max_line_length": 173,
"avg_line_length": 38.6958762886598,
"alnum_prop": 0.4747568935660051,
"repo_name": "Grimace1975/bclcontrib-web",
"id": "e38f3ddb11bd41f57689f106484a579be9a1ca53",
"size": "8604",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "System.WebEx/Web/UI+Controls/WebControls/DropDownListEx.cs",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "C#",
"bytes": "1175464"
},
{
"name": "JavaScript",
"bytes": "72820"
},
{
"name": "Shell",
"bytes": "3144"
}
],
"symlink_target": ""
} |
require 'helper'
class TestUakari < Test::Unit::TestCase
should "probably rename this file and start testing for real" do
flunk "hey buddy, you should probably rename this file and start testing for real"
end
end
| {
"content_hash": "7b19bf22da1541c1f765c95af7dd0feb",
"timestamp": "",
"source": "github",
"line_count": 7,
"max_line_length": 86,
"avg_line_length": 31.714285714285715,
"alnum_prop": 0.7567567567567568,
"repo_name": "amro/uakari",
"id": "c02693206af637df25ca51dc905cc7709c516e0a",
"size": "222",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "test/test_uakari.rb",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "Ruby",
"bytes": "8221"
}
],
"symlink_target": ""
} |
from functools import reduce
def arrayPacking(a):
return reduce(lambda x, y: x << 8 | y, a[::-1], 0)
| {
"content_hash": "2b1a6b5fb1873928a0285f3e25eb4e1b",
"timestamp": "",
"source": "github",
"line_count": 3,
"max_line_length": 54,
"avg_line_length": 35,
"alnum_prop": 0.638095238095238,
"repo_name": "RevansChen/online-judge",
"id": "6585e586ad29a0acea4041ec1520f0182665f78c",
"size": "116",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "Codefights/arcade/code-arcade/level-3/18.Array-Packing/Python/solution1.py",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "Brainfuck",
"bytes": "102"
},
{
"name": "C",
"bytes": "6829"
},
{
"name": "C#",
"bytes": "19758"
},
{
"name": "C++",
"bytes": "9439"
},
{
"name": "Clojure",
"bytes": "75"
},
{
"name": "CoffeeScript",
"bytes": "903"
},
{
"name": "Crystal",
"bytes": "52"
},
{
"name": "Dart",
"bytes": "182"
},
{
"name": "Elixir",
"bytes": "1027"
},
{
"name": "Erlang",
"bytes": "132"
},
{
"name": "F#",
"bytes": "40"
},
{
"name": "Go",
"bytes": "83"
},
{
"name": "Haskell",
"bytes": "102"
},
{
"name": "Java",
"bytes": "11057"
},
{
"name": "JavaScript",
"bytes": "44773"
},
{
"name": "Kotlin",
"bytes": "82"
},
{
"name": "Lua",
"bytes": "93"
},
{
"name": "PHP",
"bytes": "2875"
},
{
"name": "Python",
"bytes": "563400"
},
{
"name": "R",
"bytes": "265"
},
{
"name": "Ruby",
"bytes": "7171"
},
{
"name": "Rust",
"bytes": "74"
},
{
"name": "Scala",
"bytes": "84"
},
{
"name": "Shell",
"bytes": "438"
},
{
"name": "Swift",
"bytes": "6597"
},
{
"name": "TSQL",
"bytes": "3531"
},
{
"name": "TypeScript",
"bytes": "5744"
}
],
"symlink_target": ""
} |
require 'ubiquo_core'
module UbiquoVersions
class Engine < Rails::Engine
include Ubiquo::Engine::Base
initializer :load_extensions do
require 'ubiquo_versions/extensions.rb'
require 'ubiquo_versions/version.rb'
end
end
end
| {
"content_hash": "e3eae9abdc85e20aeb7a6880e118f5c1",
"timestamp": "",
"source": "github",
"line_count": 13,
"max_line_length": 45,
"avg_line_length": 19.53846153846154,
"alnum_prop": 0.7125984251968503,
"repo_name": "gnuine/ubiquo",
"id": "de293723f9f11f92090cb4b6d479635b8422eac8",
"size": "254",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "ubiquo_versions/lib/ubiquo_versions.rb",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "CSS",
"bytes": "5956"
},
{
"name": "JavaScript",
"bytes": "236340"
},
{
"name": "Ruby",
"bytes": "1478854"
}
],
"symlink_target": ""
} |
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<!--NewPage-->
<HTML>
<HEAD>
<!-- Generated by javadoc (build 1.6.0_26) on Mon May 07 13:00:01 PDT 2012 -->
<TITLE>
Uses of Class org.apache.hadoop.mapreduce.lib.partition.BinaryPartitioner (Hadoop 0.20.2-cdh3u4 API)
</TITLE>
<META NAME="date" CONTENT="2012-05-07">
<LINK REL ="stylesheet" TYPE="text/css" HREF="../../../../../../../stylesheet.css" TITLE="Style">
<SCRIPT type="text/javascript">
function windowTitle()
{
if (location.href.indexOf('is-external=true') == -1) {
parent.document.title="Uses of Class org.apache.hadoop.mapreduce.lib.partition.BinaryPartitioner (Hadoop 0.20.2-cdh3u4 API)";
}
}
</SCRIPT>
<NOSCRIPT>
</NOSCRIPT>
</HEAD>
<BODY BGCOLOR="white" onload="windowTitle();">
<HR>
<!-- ========= START OF TOP NAVBAR ======= -->
<A NAME="navbar_top"><!-- --></A>
<A HREF="#skip-navbar_top" title="Skip navigation links"></A>
<TABLE BORDER="0" WIDTH="100%" CELLPADDING="1" CELLSPACING="0" SUMMARY="">
<TR>
<TD COLSPAN=2 BGCOLOR="#EEEEFF" CLASS="NavBarCell1">
<A NAME="navbar_top_firstrow"><!-- --></A>
<TABLE BORDER="0" CELLPADDING="0" CELLSPACING="3" SUMMARY="">
<TR ALIGN="center" VALIGN="top">
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../../../overview-summary.html"><FONT CLASS="NavBarFont1"><B>Overview</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../package-summary.html"><FONT CLASS="NavBarFont1"><B>Package</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../../../org/apache/hadoop/mapreduce/lib/partition/BinaryPartitioner.html" title="class in org.apache.hadoop.mapreduce.lib.partition"><FONT CLASS="NavBarFont1"><B>Class</B></FONT></A> </TD>
<TD BGCOLOR="#FFFFFF" CLASS="NavBarCell1Rev"> <FONT CLASS="NavBarFont1Rev"><B>Use</B></FONT> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../package-tree.html"><FONT CLASS="NavBarFont1"><B>Tree</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../../../deprecated-list.html"><FONT CLASS="NavBarFont1"><B>Deprecated</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../../../index-all.html"><FONT CLASS="NavBarFont1"><B>Index</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../../../help-doc.html"><FONT CLASS="NavBarFont1"><B>Help</B></FONT></A> </TD>
</TR>
</TABLE>
</TD>
<TD ALIGN="right" VALIGN="top" ROWSPAN=3><EM>
</EM>
</TD>
</TR>
<TR>
<TD BGCOLOR="white" CLASS="NavBarCell2"><FONT SIZE="-2">
PREV
NEXT</FONT></TD>
<TD BGCOLOR="white" CLASS="NavBarCell2"><FONT SIZE="-2">
<A HREF="../../../../../../../index.html?org/apache/hadoop/mapreduce/lib/partition//class-useBinaryPartitioner.html" target="_top"><B>FRAMES</B></A>
<A HREF="BinaryPartitioner.html" target="_top"><B>NO FRAMES</B></A>
<SCRIPT type="text/javascript">
<!--
if(window==top) {
document.writeln('<A HREF="../../../../../../../allclasses-noframe.html"><B>All Classes</B></A>');
}
//-->
</SCRIPT>
<NOSCRIPT>
<A HREF="../../../../../../../allclasses-noframe.html"><B>All Classes</B></A>
</NOSCRIPT>
</FONT></TD>
</TR>
</TABLE>
<A NAME="skip-navbar_top"></A>
<!-- ========= END OF TOP NAVBAR ========= -->
<HR>
<CENTER>
<H2>
<B>Uses of Class<br>org.apache.hadoop.mapreduce.lib.partition.BinaryPartitioner</B></H2>
</CENTER>
<TABLE BORDER="1" WIDTH="100%" CELLPADDING="3" CELLSPACING="0" SUMMARY="">
<TR BGCOLOR="#CCCCFF" CLASS="TableHeadingColor">
<TH ALIGN="left" COLSPAN="2"><FONT SIZE="+2">
Packages that use <A HREF="../../../../../../../org/apache/hadoop/mapreduce/lib/partition/BinaryPartitioner.html" title="class in org.apache.hadoop.mapreduce.lib.partition">BinaryPartitioner</A></FONT></TH>
</TR>
<TR BGCOLOR="white" CLASS="TableRowColor">
<TD><A HREF="#org.apache.hadoop.mapred.lib"><B>org.apache.hadoop.mapred.lib</B></A></TD>
<TD>Library of generally useful mappers, reducers, and partitioners. </TD>
</TR>
</TABLE>
<P>
<A NAME="org.apache.hadoop.mapred.lib"><!-- --></A>
<TABLE BORDER="1" WIDTH="100%" CELLPADDING="3" CELLSPACING="0" SUMMARY="">
<TR BGCOLOR="#CCCCFF" CLASS="TableHeadingColor">
<TH ALIGN="left" COLSPAN="2"><FONT SIZE="+2">
Uses of <A HREF="../../../../../../../org/apache/hadoop/mapreduce/lib/partition/BinaryPartitioner.html" title="class in org.apache.hadoop.mapreduce.lib.partition">BinaryPartitioner</A> in <A HREF="../../../../../../../org/apache/hadoop/mapred/lib/package-summary.html">org.apache.hadoop.mapred.lib</A></FONT></TH>
</TR>
</TABLE>
<P>
<TABLE BORDER="1" WIDTH="100%" CELLPADDING="3" CELLSPACING="0" SUMMARY="">
<TR BGCOLOR="#CCCCFF" CLASS="TableSubHeadingColor">
<TH ALIGN="left" COLSPAN="2">Subclasses of <A HREF="../../../../../../../org/apache/hadoop/mapreduce/lib/partition/BinaryPartitioner.html" title="class in org.apache.hadoop.mapreduce.lib.partition">BinaryPartitioner</A> in <A HREF="../../../../../../../org/apache/hadoop/mapred/lib/package-summary.html">org.apache.hadoop.mapred.lib</A></FONT></TH>
</TR>
<TR BGCOLOR="white" CLASS="TableRowColor">
<TD ALIGN="right" VALIGN="top" WIDTH="1%"><FONT SIZE="-1">
<CODE> class</CODE></FONT></TD>
<TD><CODE><B><A HREF="../../../../../../../org/apache/hadoop/mapred/lib/BinaryPartitioner.html" title="class in org.apache.hadoop.mapred.lib">BinaryPartitioner<V></A></B></CODE>
<BR>
Partition <A HREF="../../../../../../../org/apache/hadoop/io/BinaryComparable.html" title="class in org.apache.hadoop.io"><CODE>BinaryComparable</CODE></A> keys using a configurable part of
the bytes array returned by <A HREF="../../../../../../../org/apache/hadoop/io/BinaryComparable.html#getBytes()"><CODE>BinaryComparable.getBytes()</CODE></A>.</TD>
</TR>
</TABLE>
<P>
<HR>
<!-- ======= START OF BOTTOM NAVBAR ====== -->
<A NAME="navbar_bottom"><!-- --></A>
<A HREF="#skip-navbar_bottom" title="Skip navigation links"></A>
<TABLE BORDER="0" WIDTH="100%" CELLPADDING="1" CELLSPACING="0" SUMMARY="">
<TR>
<TD COLSPAN=2 BGCOLOR="#EEEEFF" CLASS="NavBarCell1">
<A NAME="navbar_bottom_firstrow"><!-- --></A>
<TABLE BORDER="0" CELLPADDING="0" CELLSPACING="3" SUMMARY="">
<TR ALIGN="center" VALIGN="top">
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../../../overview-summary.html"><FONT CLASS="NavBarFont1"><B>Overview</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../package-summary.html"><FONT CLASS="NavBarFont1"><B>Package</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../../../org/apache/hadoop/mapreduce/lib/partition/BinaryPartitioner.html" title="class in org.apache.hadoop.mapreduce.lib.partition"><FONT CLASS="NavBarFont1"><B>Class</B></FONT></A> </TD>
<TD BGCOLOR="#FFFFFF" CLASS="NavBarCell1Rev"> <FONT CLASS="NavBarFont1Rev"><B>Use</B></FONT> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../package-tree.html"><FONT CLASS="NavBarFont1"><B>Tree</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../../../deprecated-list.html"><FONT CLASS="NavBarFont1"><B>Deprecated</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../../../index-all.html"><FONT CLASS="NavBarFont1"><B>Index</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../../../help-doc.html"><FONT CLASS="NavBarFont1"><B>Help</B></FONT></A> </TD>
</TR>
</TABLE>
</TD>
<TD ALIGN="right" VALIGN="top" ROWSPAN=3><EM>
</EM>
</TD>
</TR>
<TR>
<TD BGCOLOR="white" CLASS="NavBarCell2"><FONT SIZE="-2">
PREV
NEXT</FONT></TD>
<TD BGCOLOR="white" CLASS="NavBarCell2"><FONT SIZE="-2">
<A HREF="../../../../../../../index.html?org/apache/hadoop/mapreduce/lib/partition//class-useBinaryPartitioner.html" target="_top"><B>FRAMES</B></A>
<A HREF="BinaryPartitioner.html" target="_top"><B>NO FRAMES</B></A>
<SCRIPT type="text/javascript">
<!--
if(window==top) {
document.writeln('<A HREF="../../../../../../../allclasses-noframe.html"><B>All Classes</B></A>');
}
//-->
</SCRIPT>
<NOSCRIPT>
<A HREF="../../../../../../../allclasses-noframe.html"><B>All Classes</B></A>
</NOSCRIPT>
</FONT></TD>
</TR>
</TABLE>
<A NAME="skip-navbar_bottom"></A>
<!-- ======== END OF BOTTOM NAVBAR ======= -->
<HR>
Copyright © 2009 The Apache Software Foundation
</BODY>
</HTML>
| {
"content_hash": "b75bc43a50ea553f79bbb0824478532a",
"timestamp": "",
"source": "github",
"line_count": 181,
"max_line_length": 348,
"avg_line_length": 47.97790055248619,
"alnum_prop": 0.6310456011054814,
"repo_name": "Shmuma/hadoop",
"id": "ae7b9e7641ed404aa1dc9fe0d01eac9b940a6c81",
"size": "8684",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "docs/api/org/apache/hadoop/mapreduce/lib/partition/class-use/BinaryPartitioner.html",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "C",
"bytes": "409571"
},
{
"name": "C++",
"bytes": "403118"
},
{
"name": "CSS",
"bytes": "106092"
},
{
"name": "Java",
"bytes": "15723216"
},
{
"name": "JavaScript",
"bytes": "112012"
},
{
"name": "Objective-C",
"bytes": "119767"
},
{
"name": "PHP",
"bytes": "152555"
},
{
"name": "Perl",
"bytes": "149888"
},
{
"name": "Python",
"bytes": "1217631"
},
{
"name": "Ruby",
"bytes": "28485"
},
{
"name": "Shell",
"bytes": "1438025"
},
{
"name": "Smalltalk",
"bytes": "56562"
},
{
"name": "XSLT",
"bytes": "235231"
}
],
"symlink_target": ""
} |
using System;
using System.Collections.Generic;
using System.Configuration;
using System.Linq;
using Microsoft.Owin.Security;
using Microsoft.Owin.Security.ActiveDirectory;
using Owin;
using System.IdentityModel.Tokens;
using TodoListService.Utils;
namespace TodoListService
{
public partial class Startup
{
// For more information on configuring authentication, please visit http://go.microsoft.com/fwlink/?LinkId=301864
public void ConfigureAuth(IAppBuilder app)
{
MyTokenValidationParameters myTVPs = new MyTokenValidationParameters
{
RoleClaimType = "roles",
};
app.UseWindowsAzureActiveDirectoryBearerAuthentication(
new WindowsAzureActiveDirectoryBearerAuthenticationOptions
{
Audience = ConfigurationManager.AppSettings["ida:Audience"],
Tenant = ConfigurationManager.AppSettings["ida:Tenant"],
TokenValidationParameters = myTVPs,
TokenHandler = new MyJwtSecurityTokenHandler(),
});
}
}
}
| {
"content_hash": "be02dba84dfb75b7add3ebc8ceda30b3",
"timestamp": "",
"source": "github",
"line_count": 33,
"max_line_length": 121,
"avg_line_length": 34.45454545454545,
"alnum_prop": 0.6561125769569042,
"repo_name": "dstrockis/Bearer_WebAPI_GroupClaims",
"id": "d93cf4f73361a21ecffdc1387c60e784d79c8995",
"size": "1139",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "TodoListService/App_Start/Startup.Auth.cs",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "ASP",
"bytes": "214"
},
{
"name": "C#",
"bytes": "188058"
},
{
"name": "CSS",
"bytes": "3681"
},
{
"name": "Groff",
"bytes": "2203"
},
{
"name": "HTML",
"bytes": "10192"
},
{
"name": "JavaScript",
"bytes": "38177"
}
],
"symlink_target": ""
} |
using System;
using System.Collections.Generic;
using Microsoft.VisualStudio.TestTools.UnitTesting;
namespace NationalIdValidation.Tests
{
[TestClass]
public class EnglishNationalInsuranceUnitTests
{
private List<string> ValidIdNumbers { get; set; }
private List<string> InvalidIdNumbers { get; set; }
[TestInitialize]
public void Initialize()
{
ValidIdNumbers = new List<string>
{
"AB 12 34 56 C"
};
InvalidIdNumbers = new List<string>
{
null,
"",
"\0",
":",
"0",
"DA 00 00 00 \0",
"FA 00 00 00-",
"IA 00 00 00\u0100",
"QA 00 00 00 A\0",
"QQ 12 34 56 C",
"BO 00 00 10 A"
};
}
[TestMethod]
public void ValidatesValidNationalInsuranceIds()
{
foreach (var id in ValidIdNumbers)
{
var idObject = new EnglishNationalInsuranceId(id);
Assert.IsTrue(idObject.IsValid, $"A valid mathematically number does not validate: {id}");
}
}
[TestMethod]
public void ValidatesValidBenefitsDay()
{
foreach (var id in ValidIdNumbers)
{
var idObject = new EnglishNationalInsuranceId(id);
Assert.IsTrue(idObject.IsValid, $"A valid mathematically number does not validate: {id}");
Assert.AreEqual(DayOfWeek.Wednesday, idObject.BenefitsDay);
}
}
[TestMethod]
public void InvalidatesInvalidNationalInsuranceIds()
{
foreach (var id in InvalidIdNumbers)
{
var idObject = new EnglishNationalInsuranceId(id);
Assert.IsFalse(idObject.IsValid, $"An invalid mathematically number does validate: {id}");
}
}
}
}
| {
"content_hash": "589a46499c96f93648f040e1c22ae693",
"timestamp": "",
"source": "github",
"line_count": 67,
"max_line_length": 106,
"avg_line_length": 30.28358208955224,
"alnum_prop": 0.5140463282405126,
"repo_name": "jakobnotseth/nationalidvalidation",
"id": "b8c060dae31a5fdd7cecac63756a44618de6cd82",
"size": "2031",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "NationalIdValidation/NationalIdValidation.Tests/EnglishNationalInsuranceUnitTests.cs",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "C#",
"bytes": "89758"
}
],
"symlink_target": ""
} |
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
; Copyright (c) 2015, Intel Corporation
;
; All rights reserved.
;
; Redistribution and use in source and binary forms, with or without
; modification, are permitted provided that the following conditions are
; met:
;
; * Redistributions of source code must retain the above copyright
; notice, this list of conditions and the following disclaimer.
;
; * Redistributions in binary form must reproduce the above copyright
; notice, this list of conditions and the following disclaimer in the
; documentation and/or other materials provided with the
; distribution.
;
; * Neither the name of the Intel Corporation nor the names of its
; contributors may be used to endorse or promote products derived from
; this software without specific prior written permission.
;
;
; THIS SOFTWARE IS PROVIDED BY INTEL CORPORATION ""AS IS"" AND ANY
; EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
; IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
; PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL INTEL CORPORATION OR
; CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL,
; EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO,
; PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR
; PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF
; LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING
; NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
; SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
%define FUNC submit_job_hmac_sha_224_avx
%define SHA224
%include "mb_mgr_hmac_sha_256_submit_avx.asm"
| {
"content_hash": "a8c3f0757d04683ab46ab70ab5fc5a75",
"timestamp": "",
"source": "github",
"line_count": 39,
"max_line_length": 72,
"avg_line_length": 47.12820512820513,
"alnum_prop": 0.6926006528835691,
"repo_name": "lukego/intel-ipsec",
"id": "db62415c30be16229ae4ea9e7d71549a0d4d3cdd",
"size": "1838",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "code/avx/mb_mgr_hmac_sha_224_submit_avx.asm",
"mode": "33188",
"license": "bsd-3-clause",
"language": [
{
"name": "Assembly",
"bytes": "1115128"
},
{
"name": "C",
"bytes": "134890"
},
{
"name": "Makefile",
"bytes": "6126"
},
{
"name": "Objective-C",
"bytes": "1827"
}
],
"symlink_target": ""
} |
import * as React from "react";
import { CarbonIconProps } from "../../";
declare const Helicopter16: React.ForwardRefExoticComponent<
CarbonIconProps & React.RefAttributes<SVGSVGElement>
>;
export default Helicopter16;
| {
"content_hash": "b9d122059f731ca0897bd80f52dcd92f",
"timestamp": "",
"source": "github",
"line_count": 6,
"max_line_length": 60,
"avg_line_length": 37,
"alnum_prop": 0.7747747747747747,
"repo_name": "mcliment/DefinitelyTyped",
"id": "9c22dbfbf7f0e80806586e6e4907c8716ebf041b",
"size": "222",
"binary": false,
"copies": "2",
"ref": "refs/heads/master",
"path": "types/carbon__icons-react/lib/helicopter/16.d.ts",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "CoffeeScript",
"bytes": "15"
},
{
"name": "Protocol Buffer",
"bytes": "678"
},
{
"name": "TypeScript",
"bytes": "17214021"
}
],
"symlink_target": ""
} |
//===--- DeclSpec.h - Parsed declaration specifiers -------------*- C++ -*-===//
//
// The LLVM Compiler Infrastructure
//
// This file is distributed under the University of Illinois Open Source
// License. See LICENSE.TXT for details.
//
//===----------------------------------------------------------------------===//
///
/// \file
/// \brief This file defines the classes used to store parsed information about
/// declaration-specifiers and declarators.
///
/// \verbatim
/// static const int volatile x, *y, *(*(*z)[10])(const void *x);
/// ------------------------- - -- ---------------------------
/// declaration-specifiers \ | /
/// declarators
/// \endverbatim
///
//===----------------------------------------------------------------------===//
#ifndef LLVM_CLANG_SEMA_DECLSPEC_H
#define LLVM_CLANG_SEMA_DECLSPEC_H
#include "clang/AST/NestedNameSpecifier.h"
#include "clang/Basic/ExceptionSpecificationType.h"
#include "clang/Basic/Lambda.h"
#include "clang/Basic/OperatorKinds.h"
#include "clang/Basic/Specifiers.h"
#include "clang/Lex/Token.h"
#include "clang/Sema/AttributeList.h"
#include "clang/Sema/Ownership.h"
#include "llvm/ADT/SmallVector.h"
#include "llvm/Support/Compiler.h"
#include "llvm/Support/ErrorHandling.h"
namespace clang {
class ASTContext;
class CXXRecordDecl;
class TypeLoc;
class LangOptions;
class IdentifierInfo;
class NamespaceAliasDecl;
class NamespaceDecl;
class ObjCDeclSpec;
class Sema;
class Declarator;
struct TemplateIdAnnotation;
/// \brief Represents a C++ nested-name-specifier or a global scope specifier.
///
/// These can be in 3 states:
/// 1) Not present, identified by isEmpty()
/// 2) Present, identified by isNotEmpty()
/// 2.a) Valid, identified by isValid()
/// 2.b) Invalid, identified by isInvalid().
///
/// isSet() is deprecated because it mostly corresponded to "valid" but was
/// often used as if it meant "present".
///
/// The actual scope is described by getScopeRep().
class CXXScopeSpec {
SourceRange Range;
NestedNameSpecifierLocBuilder Builder;
public:
SourceRange getRange() const { return Range; }
void setRange(SourceRange R) { Range = R; }
void setBeginLoc(SourceLocation Loc) { Range.setBegin(Loc); }
void setEndLoc(SourceLocation Loc) { Range.setEnd(Loc); }
SourceLocation getBeginLoc() const { return Range.getBegin(); }
SourceLocation getEndLoc() const { return Range.getEnd(); }
/// \brief Retrieve the representation of the nested-name-specifier.
NestedNameSpecifier *getScopeRep() const {
return Builder.getRepresentation();
}
/// \brief Extend the current nested-name-specifier by another
/// nested-name-specifier component of the form 'type::'.
///
/// \param Context The AST context in which this nested-name-specifier
/// resides.
///
/// \param TemplateKWLoc The location of the 'template' keyword, if present.
///
/// \param TL The TypeLoc that describes the type preceding the '::'.
///
/// \param ColonColonLoc The location of the trailing '::'.
void Extend(ASTContext &Context, SourceLocation TemplateKWLoc, TypeLoc TL,
SourceLocation ColonColonLoc);
/// \brief Extend the current nested-name-specifier by another
/// nested-name-specifier component of the form 'identifier::'.
///
/// \param Context The AST context in which this nested-name-specifier
/// resides.
///
/// \param Identifier The identifier.
///
/// \param IdentifierLoc The location of the identifier.
///
/// \param ColonColonLoc The location of the trailing '::'.
void Extend(ASTContext &Context, IdentifierInfo *Identifier,
SourceLocation IdentifierLoc, SourceLocation ColonColonLoc);
/// \brief Extend the current nested-name-specifier by another
/// nested-name-specifier component of the form 'namespace::'.
///
/// \param Context The AST context in which this nested-name-specifier
/// resides.
///
/// \param Namespace The namespace.
///
/// \param NamespaceLoc The location of the namespace name.
///
/// \param ColonColonLoc The location of the trailing '::'.
void Extend(ASTContext &Context, NamespaceDecl *Namespace,
SourceLocation NamespaceLoc, SourceLocation ColonColonLoc);
/// \brief Extend the current nested-name-specifier by another
/// nested-name-specifier component of the form 'namespace-alias::'.
///
/// \param Context The AST context in which this nested-name-specifier
/// resides.
///
/// \param Alias The namespace alias.
///
/// \param AliasLoc The location of the namespace alias
/// name.
///
/// \param ColonColonLoc The location of the trailing '::'.
void Extend(ASTContext &Context, NamespaceAliasDecl *Alias,
SourceLocation AliasLoc, SourceLocation ColonColonLoc);
/// \brief Turn this (empty) nested-name-specifier into the global
/// nested-name-specifier '::'.
void MakeGlobal(ASTContext &Context, SourceLocation ColonColonLoc);
/// \brief Turns this (empty) nested-name-specifier into '__super'
/// nested-name-specifier.
///
/// \param Context The AST context in which this nested-name-specifier
/// resides.
///
/// \param RD The declaration of the class in which nested-name-specifier
/// appeared.
///
/// \param SuperLoc The location of the '__super' keyword.
/// name.
///
/// \param ColonColonLoc The location of the trailing '::'.
void MakeSuper(ASTContext &Context, CXXRecordDecl *RD,
SourceLocation SuperLoc, SourceLocation ColonColonLoc);
/// \brief Make a new nested-name-specifier from incomplete source-location
/// information.
///
/// FIXME: This routine should be used very, very rarely, in cases where we
/// need to synthesize a nested-name-specifier. Most code should instead use
/// \c Adopt() with a proper \c NestedNameSpecifierLoc.
void MakeTrivial(ASTContext &Context, NestedNameSpecifier *Qualifier,
SourceRange R);
/// \brief Adopt an existing nested-name-specifier (with source-range
/// information).
void Adopt(NestedNameSpecifierLoc Other);
/// \brief Retrieve a nested-name-specifier with location information, copied
/// into the given AST context.
///
/// \param Context The context into which this nested-name-specifier will be
/// copied.
NestedNameSpecifierLoc getWithLocInContext(ASTContext &Context) const;
/// \brief Retrieve the location of the name in the last qualifier
/// in this nested name specifier.
///
/// For example, the location of \c bar
/// in
/// \verbatim
/// \::foo::bar<0>::
/// ^~~
/// \endverbatim
SourceLocation getLastQualifierNameLoc() const;
/// No scope specifier.
bool isEmpty() const { return !Range.isValid(); }
/// A scope specifier is present, but may be valid or invalid.
bool isNotEmpty() const { return !isEmpty(); }
/// An error occurred during parsing of the scope specifier.
bool isInvalid() const { return isNotEmpty() && getScopeRep() == nullptr; }
/// A scope specifier is present, and it refers to a real scope.
bool isValid() const { return isNotEmpty() && getScopeRep() != nullptr; }
/// \brief Indicate that this nested-name-specifier is invalid.
void SetInvalid(SourceRange R) {
assert(R.isValid() && "Must have a valid source range");
if (Range.getBegin().isInvalid())
Range.setBegin(R.getBegin());
Range.setEnd(R.getEnd());
Builder.Clear();
}
/// Deprecated. Some call sites intend isNotEmpty() while others intend
/// isValid().
bool isSet() const { return getScopeRep() != nullptr; }
void clear() {
Range = SourceRange();
Builder.Clear();
}
/// \brief Retrieve the data associated with the source-location information.
char *location_data() const { return Builder.getBuffer().first; }
/// \brief Retrieve the size of the data associated with source-location
/// information.
unsigned location_size() const { return Builder.getBuffer().second; }
};
/// \brief Captures information about "declaration specifiers".
///
/// "Declaration specifiers" encompasses storage-class-specifiers,
/// type-specifiers, type-qualifiers, and function-specifiers.
class DeclSpec {
public:
/// \brief storage-class-specifier
/// \note The order of these enumerators is important for diagnostics.
enum SCS {
SCS_unspecified = 0,
SCS_typedef,
SCS_extern,
SCS_static,
SCS_auto,
SCS_register,
SCS_private_extern,
SCS_mutable
};
// Import thread storage class specifier enumeration and constants.
// These can be combined with SCS_extern and SCS_static.
typedef ThreadStorageClassSpecifier TSCS;
static const TSCS TSCS_unspecified = clang::TSCS_unspecified;
static const TSCS TSCS___thread = clang::TSCS___thread;
static const TSCS TSCS_thread_local = clang::TSCS_thread_local;
static const TSCS TSCS__Thread_local = clang::TSCS__Thread_local;
// Import type specifier width enumeration and constants.
typedef TypeSpecifierWidth TSW;
static const TSW TSW_unspecified = clang::TSW_unspecified;
static const TSW TSW_short = clang::TSW_short;
static const TSW TSW_long = clang::TSW_long;
static const TSW TSW_longlong = clang::TSW_longlong;
enum TSC {
TSC_unspecified,
TSC_imaginary,
TSC_complex
};
// Import type specifier sign enumeration and constants.
typedef TypeSpecifierSign TSS;
static const TSS TSS_unspecified = clang::TSS_unspecified;
static const TSS TSS_signed = clang::TSS_signed;
static const TSS TSS_unsigned = clang::TSS_unsigned;
// Import type specifier type enumeration and constants.
typedef TypeSpecifierType TST;
static const TST TST_unspecified = clang::TST_unspecified;
static const TST TST_void = clang::TST_void;
static const TST TST_char = clang::TST_char;
static const TST TST_wchar = clang::TST_wchar;
static const TST TST_char16 = clang::TST_char16;
static const TST TST_char32 = clang::TST_char32;
static const TST TST_int = clang::TST_int;
static const TST TST_int128 = clang::TST_int128;
static const TST TST_half = clang::TST_half;
static const TST TST_float = clang::TST_float;
static const TST TST_double = clang::TST_double;
static const TST TST_bool = clang::TST_bool;
static const TST TST_decimal32 = clang::TST_decimal32;
static const TST TST_decimal64 = clang::TST_decimal64;
static const TST TST_decimal128 = clang::TST_decimal128;
static const TST TST_enum = clang::TST_enum;
static const TST TST_union = clang::TST_union;
static const TST TST_struct = clang::TST_struct;
static const TST TST_interface = clang::TST_interface;
static const TST TST_class = clang::TST_class;
static const TST TST_typename = clang::TST_typename;
static const TST TST_typeofType = clang::TST_typeofType;
static const TST TST_typeofExpr = clang::TST_typeofExpr;
static const TST TST_decltype = clang::TST_decltype;
static const TST TST_decltype_auto = clang::TST_decltype_auto;
static const TST TST_underlyingType = clang::TST_underlyingType;
static const TST TST_auto = clang::TST_auto;
static const TST TST_auto_type = clang::TST_auto_type;
static const TST TST_unknown_anytype = clang::TST_unknown_anytype;
static const TST TST_atomic = clang::TST_atomic;
static const TST TST_error = clang::TST_error;
// type-qualifiers
enum TQ { // NOTE: These flags must be kept in sync with Qualifiers::TQ.
TQ_unspecified = 0,
TQ_const = 1,
TQ_restrict = 2,
TQ_volatile = 4,
// This has no corresponding Qualifiers::TQ value, because it's not treated
// as a qualifier in our type system.
TQ_atomic = 8
};
/// ParsedSpecifiers - Flags to query which specifiers were applied. This is
/// returned by getParsedSpecifiers.
enum ParsedSpecifiers {
PQ_None = 0,
PQ_StorageClassSpecifier = 1,
PQ_TypeSpecifier = 2,
PQ_TypeQualifier = 4,
PQ_FunctionSpecifier = 8
};
private:
// storage-class-specifier
/*SCS*/unsigned StorageClassSpec : 3;
/*TSCS*/unsigned ThreadStorageClassSpec : 2;
unsigned SCS_extern_in_linkage_spec : 1;
// type-specifier
/*TSW*/unsigned TypeSpecWidth : 2;
/*TSC*/unsigned TypeSpecComplex : 2;
/*TSS*/unsigned TypeSpecSign : 2;
/*TST*/unsigned TypeSpecType : 6;
unsigned TypeAltiVecVector : 1;
unsigned TypeAltiVecPixel : 1;
unsigned TypeAltiVecBool : 1;
unsigned TypeSpecOwned : 1;
unsigned TypeSpecPipe : 1;
// type-qualifiers
unsigned TypeQualifiers : 4; // Bitwise OR of TQ.
// function-specifier
unsigned FS_inline_specified : 1;
unsigned FS_forceinline_specified: 1;
unsigned FS_virtual_specified : 1;
unsigned FS_explicit_specified : 1;
unsigned FS_noreturn_specified : 1;
// friend-specifier
unsigned Friend_specified : 1;
// constexpr-specifier
unsigned Constexpr_specified : 1;
// concept-specifier
unsigned Concept_specified : 1;
union {
UnionParsedType TypeRep;
Decl *DeclRep;
Expr *ExprRep;
};
// attributes.
ParsedAttributes Attrs;
// Scope specifier for the type spec, if applicable.
CXXScopeSpec TypeScope;
// SourceLocation info. These are null if the item wasn't specified or if
// the setting was synthesized.
SourceRange Range;
SourceLocation StorageClassSpecLoc, ThreadStorageClassSpecLoc;
SourceLocation TSWLoc, TSCLoc, TSSLoc, TSTLoc, AltiVecLoc;
/// TSTNameLoc - If TypeSpecType is any of class, enum, struct, union,
/// typename, then this is the location of the named type (if present);
/// otherwise, it is the same as TSTLoc. Hence, the pair TSTLoc and
/// TSTNameLoc provides source range info for tag types.
SourceLocation TSTNameLoc;
SourceRange TypeofParensRange;
SourceLocation TQ_constLoc, TQ_restrictLoc, TQ_volatileLoc, TQ_atomicLoc;
SourceLocation FS_inlineLoc, FS_virtualLoc, FS_explicitLoc, FS_noreturnLoc;
SourceLocation FS_forceinlineLoc;
SourceLocation FriendLoc, ModulePrivateLoc, ConstexprLoc, ConceptLoc;
SourceLocation TQ_pipeLoc;
WrittenBuiltinSpecs writtenBS;
void SaveWrittenBuiltinSpecs();
ObjCDeclSpec *ObjCQualifiers;
static bool isTypeRep(TST T) {
return (T == TST_typename || T == TST_typeofType ||
T == TST_underlyingType || T == TST_atomic);
}
static bool isExprRep(TST T) {
return (T == TST_typeofExpr || T == TST_decltype);
}
DeclSpec(const DeclSpec &) = delete;
void operator=(const DeclSpec &) = delete;
public:
static bool isDeclRep(TST T) {
return (T == TST_enum || T == TST_struct ||
T == TST_interface || T == TST_union ||
T == TST_class);
}
DeclSpec(AttributeFactory &attrFactory)
: StorageClassSpec(SCS_unspecified),
ThreadStorageClassSpec(TSCS_unspecified),
SCS_extern_in_linkage_spec(false),
TypeSpecWidth(TSW_unspecified),
TypeSpecComplex(TSC_unspecified),
TypeSpecSign(TSS_unspecified),
TypeSpecType(TST_unspecified),
TypeAltiVecVector(false),
TypeAltiVecPixel(false),
TypeAltiVecBool(false),
TypeSpecOwned(false),
TypeSpecPipe(false),
TypeQualifiers(TQ_unspecified),
FS_inline_specified(false),
FS_forceinline_specified(false),
FS_virtual_specified(false),
FS_explicit_specified(false),
FS_noreturn_specified(false),
Friend_specified(false),
Constexpr_specified(false),
Concept_specified(false),
Attrs(attrFactory),
writtenBS(),
ObjCQualifiers(nullptr) {
}
// storage-class-specifier
SCS getStorageClassSpec() const { return (SCS)StorageClassSpec; }
TSCS getThreadStorageClassSpec() const {
return (TSCS)ThreadStorageClassSpec;
}
bool isExternInLinkageSpec() const { return SCS_extern_in_linkage_spec; }
void setExternInLinkageSpec(bool Value) {
SCS_extern_in_linkage_spec = Value;
}
SourceLocation getStorageClassSpecLoc() const { return StorageClassSpecLoc; }
SourceLocation getThreadStorageClassSpecLoc() const {
return ThreadStorageClassSpecLoc;
}
void ClearStorageClassSpecs() {
StorageClassSpec = DeclSpec::SCS_unspecified;
ThreadStorageClassSpec = DeclSpec::TSCS_unspecified;
SCS_extern_in_linkage_spec = false;
StorageClassSpecLoc = SourceLocation();
ThreadStorageClassSpecLoc = SourceLocation();
}
void ClearTypeSpecType() {
TypeSpecType = DeclSpec::TST_unspecified;
TypeSpecOwned = false;
TSTLoc = SourceLocation();
}
// type-specifier
TSW getTypeSpecWidth() const { return (TSW)TypeSpecWidth; }
TSC getTypeSpecComplex() const { return (TSC)TypeSpecComplex; }
TSS getTypeSpecSign() const { return (TSS)TypeSpecSign; }
TST getTypeSpecType() const { return (TST)TypeSpecType; }
bool isTypeAltiVecVector() const { return TypeAltiVecVector; }
bool isTypeAltiVecPixel() const { return TypeAltiVecPixel; }
bool isTypeAltiVecBool() const { return TypeAltiVecBool; }
bool isTypeSpecOwned() const { return TypeSpecOwned; }
bool isTypeRep() const { return isTypeRep((TST) TypeSpecType); }
bool isTypeSpecPipe() const { return TypeSpecPipe; }
ParsedType getRepAsType() const {
assert(isTypeRep((TST) TypeSpecType) && "DeclSpec does not store a type");
return TypeRep;
}
Decl *getRepAsDecl() const {
assert(isDeclRep((TST) TypeSpecType) && "DeclSpec does not store a decl");
return DeclRep;
}
Expr *getRepAsExpr() const {
assert(isExprRep((TST) TypeSpecType) && "DeclSpec does not store an expr");
return ExprRep;
}
CXXScopeSpec &getTypeSpecScope() { return TypeScope; }
const CXXScopeSpec &getTypeSpecScope() const { return TypeScope; }
SourceRange getSourceRange() const LLVM_READONLY { return Range; }
SourceLocation getLocStart() const LLVM_READONLY { return Range.getBegin(); }
SourceLocation getLocEnd() const LLVM_READONLY { return Range.getEnd(); }
SourceLocation getTypeSpecWidthLoc() const { return TSWLoc; }
SourceLocation getTypeSpecComplexLoc() const { return TSCLoc; }
SourceLocation getTypeSpecSignLoc() const { return TSSLoc; }
SourceLocation getTypeSpecTypeLoc() const { return TSTLoc; }
SourceLocation getAltiVecLoc() const { return AltiVecLoc; }
SourceLocation getTypeSpecTypeNameLoc() const {
assert(isDeclRep((TST) TypeSpecType) || TypeSpecType == TST_typename);
return TSTNameLoc;
}
SourceRange getTypeofParensRange() const { return TypeofParensRange; }
void setTypeofParensRange(SourceRange range) { TypeofParensRange = range; }
bool containsPlaceholderType() const {
return (TypeSpecType == TST_auto || TypeSpecType == TST_auto_type ||
TypeSpecType == TST_decltype_auto);
}
bool hasTagDefinition() const;
/// \brief Turn a type-specifier-type into a string like "_Bool" or "union".
static const char *getSpecifierName(DeclSpec::TST T,
const PrintingPolicy &Policy);
static const char *getSpecifierName(DeclSpec::TQ Q);
static const char *getSpecifierName(DeclSpec::TSS S);
static const char *getSpecifierName(DeclSpec::TSC C);
static const char *getSpecifierName(DeclSpec::TSW W);
static const char *getSpecifierName(DeclSpec::SCS S);
static const char *getSpecifierName(DeclSpec::TSCS S);
// type-qualifiers
/// getTypeQualifiers - Return a set of TQs.
unsigned getTypeQualifiers() const { return TypeQualifiers; }
SourceLocation getConstSpecLoc() const { return TQ_constLoc; }
SourceLocation getRestrictSpecLoc() const { return TQ_restrictLoc; }
SourceLocation getVolatileSpecLoc() const { return TQ_volatileLoc; }
SourceLocation getAtomicSpecLoc() const { return TQ_atomicLoc; }
SourceLocation getPipeLoc() const { return TQ_pipeLoc; }
/// \brief Clear out all of the type qualifiers.
void ClearTypeQualifiers() {
TypeQualifiers = 0;
TQ_constLoc = SourceLocation();
TQ_restrictLoc = SourceLocation();
TQ_volatileLoc = SourceLocation();
TQ_atomicLoc = SourceLocation();
TQ_pipeLoc = SourceLocation();
}
// function-specifier
bool isInlineSpecified() const {
return FS_inline_specified | FS_forceinline_specified;
}
SourceLocation getInlineSpecLoc() const {
return FS_inline_specified ? FS_inlineLoc : FS_forceinlineLoc;
}
bool isVirtualSpecified() const { return FS_virtual_specified; }
SourceLocation getVirtualSpecLoc() const { return FS_virtualLoc; }
bool isExplicitSpecified() const { return FS_explicit_specified; }
SourceLocation getExplicitSpecLoc() const { return FS_explicitLoc; }
bool isNoreturnSpecified() const { return FS_noreturn_specified; }
SourceLocation getNoreturnSpecLoc() const { return FS_noreturnLoc; }
void ClearFunctionSpecs() {
FS_inline_specified = false;
FS_inlineLoc = SourceLocation();
FS_forceinline_specified = false;
FS_forceinlineLoc = SourceLocation();
FS_virtual_specified = false;
FS_virtualLoc = SourceLocation();
FS_explicit_specified = false;
FS_explicitLoc = SourceLocation();
FS_noreturn_specified = false;
FS_noreturnLoc = SourceLocation();
}
/// \brief Return true if any type-specifier has been found.
bool hasTypeSpecifier() const {
return getTypeSpecType() != DeclSpec::TST_unspecified ||
getTypeSpecWidth() != DeclSpec::TSW_unspecified ||
getTypeSpecComplex() != DeclSpec::TSC_unspecified ||
getTypeSpecSign() != DeclSpec::TSS_unspecified;
}
/// \brief Return a bitmask of which flavors of specifiers this
/// DeclSpec includes.
unsigned getParsedSpecifiers() const;
/// isEmpty - Return true if this declaration specifier is completely empty:
/// no tokens were parsed in the production of it.
bool isEmpty() const {
return getParsedSpecifiers() == DeclSpec::PQ_None;
}
void SetRangeStart(SourceLocation Loc) { Range.setBegin(Loc); }
void SetRangeEnd(SourceLocation Loc) { Range.setEnd(Loc); }
/// These methods set the specified attribute of the DeclSpec and
/// return false if there was no error. If an error occurs (for
/// example, if we tried to set "auto" on a spec with "extern"
/// already set), they return true and set PrevSpec and DiagID
/// such that
/// Diag(Loc, DiagID) << PrevSpec;
/// will yield a useful result.
///
/// TODO: use a more general approach that still allows these
/// diagnostics to be ignored when desired.
bool SetStorageClassSpec(Sema &S, SCS SC, SourceLocation Loc,
const char *&PrevSpec, unsigned &DiagID,
const PrintingPolicy &Policy);
bool SetStorageClassSpecThread(TSCS TSC, SourceLocation Loc,
const char *&PrevSpec, unsigned &DiagID);
bool SetTypeSpecWidth(TSW W, SourceLocation Loc, const char *&PrevSpec,
unsigned &DiagID, const PrintingPolicy &Policy);
bool SetTypeSpecComplex(TSC C, SourceLocation Loc, const char *&PrevSpec,
unsigned &DiagID);
bool SetTypeSpecSign(TSS S, SourceLocation Loc, const char *&PrevSpec,
unsigned &DiagID);
bool SetTypeSpecType(TST T, SourceLocation Loc, const char *&PrevSpec,
unsigned &DiagID, const PrintingPolicy &Policy);
bool SetTypeSpecType(TST T, SourceLocation Loc, const char *&PrevSpec,
unsigned &DiagID, ParsedType Rep,
const PrintingPolicy &Policy);
bool SetTypeSpecType(TST T, SourceLocation Loc, const char *&PrevSpec,
unsigned &DiagID, Decl *Rep, bool Owned,
const PrintingPolicy &Policy);
bool SetTypeSpecType(TST T, SourceLocation TagKwLoc,
SourceLocation TagNameLoc, const char *&PrevSpec,
unsigned &DiagID, ParsedType Rep,
const PrintingPolicy &Policy);
bool SetTypeSpecType(TST T, SourceLocation TagKwLoc,
SourceLocation TagNameLoc, const char *&PrevSpec,
unsigned &DiagID, Decl *Rep, bool Owned,
const PrintingPolicy &Policy);
bool SetTypeSpecType(TST T, SourceLocation Loc, const char *&PrevSpec,
unsigned &DiagID, Expr *Rep,
const PrintingPolicy &policy);
bool SetTypeAltiVecVector(bool isAltiVecVector, SourceLocation Loc,
const char *&PrevSpec, unsigned &DiagID,
const PrintingPolicy &Policy);
bool SetTypeAltiVecPixel(bool isAltiVecPixel, SourceLocation Loc,
const char *&PrevSpec, unsigned &DiagID,
const PrintingPolicy &Policy);
bool SetTypeAltiVecBool(bool isAltiVecBool, SourceLocation Loc,
const char *&PrevSpec, unsigned &DiagID,
const PrintingPolicy &Policy);
bool SetTypePipe(bool isPipe, SourceLocation Loc,
const char *&PrevSpec, unsigned &DiagID,
const PrintingPolicy &Policy);
bool SetTypeSpecError();
void UpdateDeclRep(Decl *Rep) {
assert(isDeclRep((TST) TypeSpecType));
DeclRep = Rep;
}
void UpdateTypeRep(ParsedType Rep) {
assert(isTypeRep((TST) TypeSpecType));
TypeRep = Rep;
}
void UpdateExprRep(Expr *Rep) {
assert(isExprRep((TST) TypeSpecType));
ExprRep = Rep;
}
bool SetTypeQual(TQ T, SourceLocation Loc, const char *&PrevSpec,
unsigned &DiagID, const LangOptions &Lang);
bool setFunctionSpecInline(SourceLocation Loc, const char *&PrevSpec,
unsigned &DiagID);
bool setFunctionSpecForceInline(SourceLocation Loc, const char *&PrevSpec,
unsigned &DiagID);
bool setFunctionSpecVirtual(SourceLocation Loc, const char *&PrevSpec,
unsigned &DiagID);
bool setFunctionSpecExplicit(SourceLocation Loc, const char *&PrevSpec,
unsigned &DiagID);
bool setFunctionSpecNoreturn(SourceLocation Loc, const char *&PrevSpec,
unsigned &DiagID);
bool SetFriendSpec(SourceLocation Loc, const char *&PrevSpec,
unsigned &DiagID);
bool setModulePrivateSpec(SourceLocation Loc, const char *&PrevSpec,
unsigned &DiagID);
bool SetConstexprSpec(SourceLocation Loc, const char *&PrevSpec,
unsigned &DiagID);
bool SetConceptSpec(SourceLocation Loc, const char *&PrevSpec,
unsigned &DiagID);
bool isFriendSpecified() const { return Friend_specified; }
SourceLocation getFriendSpecLoc() const { return FriendLoc; }
bool isModulePrivateSpecified() const { return ModulePrivateLoc.isValid(); }
SourceLocation getModulePrivateSpecLoc() const { return ModulePrivateLoc; }
bool isConstexprSpecified() const { return Constexpr_specified; }
SourceLocation getConstexprSpecLoc() const { return ConstexprLoc; }
bool isConceptSpecified() const { return Concept_specified; }
SourceLocation getConceptSpecLoc() const { return ConceptLoc; }
void ClearConstexprSpec() {
Constexpr_specified = false;
ConstexprLoc = SourceLocation();
}
void ClearConceptSpec() {
Concept_specified = false;
ConceptLoc = SourceLocation();
}
AttributePool &getAttributePool() const {
return Attrs.getPool();
}
/// \brief Concatenates two attribute lists.
///
/// The GCC attribute syntax allows for the following:
///
/// \code
/// short __attribute__(( unused, deprecated ))
/// int __attribute__(( may_alias, aligned(16) )) var;
/// \endcode
///
/// This declares 4 attributes using 2 lists. The following syntax is
/// also allowed and equivalent to the previous declaration.
///
/// \code
/// short __attribute__((unused)) __attribute__((deprecated))
/// int __attribute__((may_alias)) __attribute__((aligned(16))) var;
/// \endcode
///
void addAttributes(AttributeList *AL) {
Attrs.addAll(AL);
}
bool hasAttributes() const { return !Attrs.empty(); }
ParsedAttributes &getAttributes() { return Attrs; }
const ParsedAttributes &getAttributes() const { return Attrs; }
void takeAttributesFrom(ParsedAttributes &attrs) {
Attrs.takeAllFrom(attrs);
}
/// Finish - This does final analysis of the declspec, issuing diagnostics for
/// things like "_Imaginary" (lacking an FP type). After calling this method,
/// DeclSpec is guaranteed self-consistent, even if an error occurred.
void Finish(Sema &S, const PrintingPolicy &Policy);
const WrittenBuiltinSpecs& getWrittenBuiltinSpecs() const {
return writtenBS;
}
ObjCDeclSpec *getObjCQualifiers() const { return ObjCQualifiers; }
void setObjCQualifiers(ObjCDeclSpec *quals) { ObjCQualifiers = quals; }
/// \brief Checks if this DeclSpec can stand alone, without a Declarator.
///
/// Only tag declspecs can stand alone.
bool isMissingDeclaratorOk();
};
/// \brief Captures information about "declaration specifiers" specific to
/// Objective-C.
class ObjCDeclSpec {
public:
/// ObjCDeclQualifier - Qualifier used on types in method
/// declarations. Not all combinations are sensible. Parameters
/// can be one of { in, out, inout } with one of { bycopy, byref }.
/// Returns can either be { oneway } or not.
///
/// This should be kept in sync with Decl::ObjCDeclQualifier.
enum ObjCDeclQualifier {
DQ_None = 0x0,
DQ_In = 0x1,
DQ_Inout = 0x2,
DQ_Out = 0x4,
DQ_Bycopy = 0x8,
DQ_Byref = 0x10,
DQ_Oneway = 0x20,
DQ_CSNullability = 0x40
};
/// PropertyAttributeKind - list of property attributes.
enum ObjCPropertyAttributeKind {
DQ_PR_noattr = 0x0,
DQ_PR_readonly = 0x01,
DQ_PR_getter = 0x02,
DQ_PR_assign = 0x04,
DQ_PR_readwrite = 0x08,
DQ_PR_retain = 0x10,
DQ_PR_copy = 0x20,
DQ_PR_nonatomic = 0x40,
DQ_PR_setter = 0x80,
DQ_PR_atomic = 0x100,
DQ_PR_weak = 0x200,
DQ_PR_strong = 0x400,
DQ_PR_unsafe_unretained = 0x800,
DQ_PR_nullability = 0x1000,
DQ_PR_null_resettable = 0x2000
};
ObjCDeclSpec()
: objcDeclQualifier(DQ_None), PropertyAttributes(DQ_PR_noattr),
Nullability(0), GetterName(nullptr), SetterName(nullptr) { }
ObjCDeclQualifier getObjCDeclQualifier() const { return objcDeclQualifier; }
void setObjCDeclQualifier(ObjCDeclQualifier DQVal) {
objcDeclQualifier = (ObjCDeclQualifier) (objcDeclQualifier | DQVal);
}
void clearObjCDeclQualifier(ObjCDeclQualifier DQVal) {
objcDeclQualifier = (ObjCDeclQualifier) (objcDeclQualifier & ~DQVal);
}
ObjCPropertyAttributeKind getPropertyAttributes() const {
return ObjCPropertyAttributeKind(PropertyAttributes);
}
void setPropertyAttributes(ObjCPropertyAttributeKind PRVal) {
PropertyAttributes =
(ObjCPropertyAttributeKind)(PropertyAttributes | PRVal);
}
NullabilityKind getNullability() const {
assert(((getObjCDeclQualifier() & DQ_CSNullability) ||
(getPropertyAttributes() & DQ_PR_nullability)) &&
"Objective-C declspec doesn't have nullability");
return static_cast<NullabilityKind>(Nullability);
}
SourceLocation getNullabilityLoc() const {
assert(((getObjCDeclQualifier() & DQ_CSNullability) ||
(getPropertyAttributes() & DQ_PR_nullability)) &&
"Objective-C declspec doesn't have nullability");
return NullabilityLoc;
}
void setNullability(SourceLocation loc, NullabilityKind kind) {
assert(((getObjCDeclQualifier() & DQ_CSNullability) ||
(getPropertyAttributes() & DQ_PR_nullability)) &&
"Set the nullability declspec or property attribute first");
Nullability = static_cast<unsigned>(kind);
NullabilityLoc = loc;
}
const IdentifierInfo *getGetterName() const { return GetterName; }
IdentifierInfo *getGetterName() { return GetterName; }
void setGetterName(IdentifierInfo *name) { GetterName = name; }
const IdentifierInfo *getSetterName() const { return SetterName; }
IdentifierInfo *getSetterName() { return SetterName; }
void setSetterName(IdentifierInfo *name) { SetterName = name; }
private:
// FIXME: These two are unrelated and mutually exclusive. So perhaps
// we can put them in a union to reflect their mutual exclusivity
// (space saving is negligible).
ObjCDeclQualifier objcDeclQualifier : 7;
// NOTE: VC++ treats enums as signed, avoid using ObjCPropertyAttributeKind
unsigned PropertyAttributes : 14;
unsigned Nullability : 2;
SourceLocation NullabilityLoc;
IdentifierInfo *GetterName; // getter name or NULL if no getter
IdentifierInfo *SetterName; // setter name or NULL if no setter
};
/// \brief Represents a C++ unqualified-id that has been parsed.
class UnqualifiedId {
private:
UnqualifiedId(const UnqualifiedId &Other) = delete;
const UnqualifiedId &operator=(const UnqualifiedId &) = delete;
public:
/// \brief Describes the kind of unqualified-id parsed.
enum IdKind {
/// \brief An identifier.
IK_Identifier,
/// \brief An overloaded operator name, e.g., operator+.
IK_OperatorFunctionId,
/// \brief A conversion function name, e.g., operator int.
IK_ConversionFunctionId,
/// \brief A user-defined literal name, e.g., operator "" _i.
IK_LiteralOperatorId,
/// \brief A constructor name.
IK_ConstructorName,
/// \brief A constructor named via a template-id.
IK_ConstructorTemplateId,
/// \brief A destructor name.
IK_DestructorName,
/// \brief A template-id, e.g., f<int>.
IK_TemplateId,
/// \brief An implicit 'self' parameter
IK_ImplicitSelfParam
} Kind;
struct OFI {
/// \brief The kind of overloaded operator.
OverloadedOperatorKind Operator;
/// \brief The source locations of the individual tokens that name
/// the operator, e.g., the "new", "[", and "]" tokens in
/// operator new [].
///
/// Different operators have different numbers of tokens in their name,
/// up to three. Any remaining source locations in this array will be
/// set to an invalid value for operators with fewer than three tokens.
unsigned SymbolLocations[3];
};
/// \brief Anonymous union that holds extra data associated with the
/// parsed unqualified-id.
union {
/// \brief When Kind == IK_Identifier, the parsed identifier, or when Kind
/// == IK_UserLiteralId, the identifier suffix.
IdentifierInfo *Identifier;
/// \brief When Kind == IK_OperatorFunctionId, the overloaded operator
/// that we parsed.
struct OFI OperatorFunctionId;
/// \brief When Kind == IK_ConversionFunctionId, the type that the
/// conversion function names.
UnionParsedType ConversionFunctionId;
/// \brief When Kind == IK_ConstructorName, the class-name of the type
/// whose constructor is being referenced.
UnionParsedType ConstructorName;
/// \brief When Kind == IK_DestructorName, the type referred to by the
/// class-name.
UnionParsedType DestructorName;
/// \brief When Kind == IK_TemplateId or IK_ConstructorTemplateId,
/// the template-id annotation that contains the template name and
/// template arguments.
TemplateIdAnnotation *TemplateId;
};
/// \brief The location of the first token that describes this unqualified-id,
/// which will be the location of the identifier, "operator" keyword,
/// tilde (for a destructor), or the template name of a template-id.
SourceLocation StartLocation;
/// \brief The location of the last token that describes this unqualified-id.
SourceLocation EndLocation;
UnqualifiedId() : Kind(IK_Identifier), Identifier(nullptr) { }
/// \brief Clear out this unqualified-id, setting it to default (invalid)
/// state.
void clear() {
Kind = IK_Identifier;
Identifier = nullptr;
StartLocation = SourceLocation();
EndLocation = SourceLocation();
}
/// \brief Determine whether this unqualified-id refers to a valid name.
bool isValid() const { return StartLocation.isValid(); }
/// \brief Determine whether this unqualified-id refers to an invalid name.
bool isInvalid() const { return !isValid(); }
/// \brief Determine what kind of name we have.
IdKind getKind() const { return Kind; }
void setKind(IdKind kind) { Kind = kind; }
/// \brief Specify that this unqualified-id was parsed as an identifier.
///
/// \param Id the parsed identifier.
/// \param IdLoc the location of the parsed identifier.
void setIdentifier(const IdentifierInfo *Id, SourceLocation IdLoc) {
Kind = IK_Identifier;
Identifier = const_cast<IdentifierInfo *>(Id);
StartLocation = EndLocation = IdLoc;
}
/// \brief Specify that this unqualified-id was parsed as an
/// operator-function-id.
///
/// \param OperatorLoc the location of the 'operator' keyword.
///
/// \param Op the overloaded operator.
///
/// \param SymbolLocations the locations of the individual operator symbols
/// in the operator.
void setOperatorFunctionId(SourceLocation OperatorLoc,
OverloadedOperatorKind Op,
SourceLocation SymbolLocations[3]);
/// \brief Specify that this unqualified-id was parsed as a
/// conversion-function-id.
///
/// \param OperatorLoc the location of the 'operator' keyword.
///
/// \param Ty the type to which this conversion function is converting.
///
/// \param EndLoc the location of the last token that makes up the type name.
void setConversionFunctionId(SourceLocation OperatorLoc,
ParsedType Ty,
SourceLocation EndLoc) {
Kind = IK_ConversionFunctionId;
StartLocation = OperatorLoc;
EndLocation = EndLoc;
ConversionFunctionId = Ty;
}
/// \brief Specific that this unqualified-id was parsed as a
/// literal-operator-id.
///
/// \param Id the parsed identifier.
///
/// \param OpLoc the location of the 'operator' keyword.
///
/// \param IdLoc the location of the identifier.
void setLiteralOperatorId(const IdentifierInfo *Id, SourceLocation OpLoc,
SourceLocation IdLoc) {
Kind = IK_LiteralOperatorId;
Identifier = const_cast<IdentifierInfo *>(Id);
StartLocation = OpLoc;
EndLocation = IdLoc;
}
/// \brief Specify that this unqualified-id was parsed as a constructor name.
///
/// \param ClassType the class type referred to by the constructor name.
///
/// \param ClassNameLoc the location of the class name.
///
/// \param EndLoc the location of the last token that makes up the type name.
void setConstructorName(ParsedType ClassType,
SourceLocation ClassNameLoc,
SourceLocation EndLoc) {
Kind = IK_ConstructorName;
StartLocation = ClassNameLoc;
EndLocation = EndLoc;
ConstructorName = ClassType;
}
/// \brief Specify that this unqualified-id was parsed as a
/// template-id that names a constructor.
///
/// \param TemplateId the template-id annotation that describes the parsed
/// template-id. This UnqualifiedId instance will take ownership of the
/// \p TemplateId and will free it on destruction.
void setConstructorTemplateId(TemplateIdAnnotation *TemplateId);
/// \brief Specify that this unqualified-id was parsed as a destructor name.
///
/// \param TildeLoc the location of the '~' that introduces the destructor
/// name.
///
/// \param ClassType the name of the class referred to by the destructor name.
void setDestructorName(SourceLocation TildeLoc,
ParsedType ClassType,
SourceLocation EndLoc) {
Kind = IK_DestructorName;
StartLocation = TildeLoc;
EndLocation = EndLoc;
DestructorName = ClassType;
}
/// \brief Specify that this unqualified-id was parsed as a template-id.
///
/// \param TemplateId the template-id annotation that describes the parsed
/// template-id. This UnqualifiedId instance will take ownership of the
/// \p TemplateId and will free it on destruction.
void setTemplateId(TemplateIdAnnotation *TemplateId);
/// \brief Return the source range that covers this unqualified-id.
SourceRange getSourceRange() const LLVM_READONLY {
return SourceRange(StartLocation, EndLocation);
}
SourceLocation getLocStart() const LLVM_READONLY { return StartLocation; }
SourceLocation getLocEnd() const LLVM_READONLY { return EndLocation; }
};
/// \brief A set of tokens that has been cached for later parsing.
typedef SmallVector<Token, 4> CachedTokens;
/// \brief One instance of this struct is used for each type in a
/// declarator that is parsed.
///
/// This is intended to be a small value object.
struct DeclaratorChunk {
enum {
Pointer, Reference, Array, Function, BlockPointer, MemberPointer, Paren, Pipe
} Kind;
/// Loc - The place where this type was defined.
SourceLocation Loc;
/// EndLoc - If valid, the place where this chunck ends.
SourceLocation EndLoc;
SourceRange getSourceRange() const {
if (EndLoc.isInvalid())
return SourceRange(Loc, Loc);
return SourceRange(Loc, EndLoc);
}
struct TypeInfoCommon {
AttributeList *AttrList;
};
struct PointerTypeInfo : TypeInfoCommon {
/// The type qualifiers: const/volatile/restrict/atomic.
unsigned TypeQuals : 4;
/// The location of the const-qualifier, if any.
unsigned ConstQualLoc;
/// The location of the volatile-qualifier, if any.
unsigned VolatileQualLoc;
/// The location of the restrict-qualifier, if any.
unsigned RestrictQualLoc;
/// The location of the _Atomic-qualifier, if any.
unsigned AtomicQualLoc;
void destroy() {
}
};
struct ReferenceTypeInfo : TypeInfoCommon {
/// The type qualifier: restrict. [GNU] C++ extension
bool HasRestrict : 1;
/// True if this is an lvalue reference, false if it's an rvalue reference.
bool LValueRef : 1;
void destroy() {
}
};
struct ArrayTypeInfo : TypeInfoCommon {
/// The type qualifiers for the array: const/volatile/restrict/_Atomic.
unsigned TypeQuals : 4;
/// True if this dimension included the 'static' keyword.
bool hasStatic : 1;
/// True if this dimension was [*]. In this case, NumElts is null.
bool isStar : 1;
/// This is the size of the array, or null if [] or [*] was specified.
/// Since the parser is multi-purpose, and we don't want to impose a root
/// expression class on all clients, NumElts is untyped.
Expr *NumElts;
void destroy() {}
};
/// ParamInfo - An array of paraminfo objects is allocated whenever a function
/// declarator is parsed. There are two interesting styles of parameters
/// here:
/// K&R-style identifier lists and parameter type lists. K&R-style identifier
/// lists will have information about the identifier, but no type information.
/// Parameter type lists will have type info (if the actions module provides
/// it), but may have null identifier info: e.g. for 'void foo(int X, int)'.
struct ParamInfo {
IdentifierInfo *Ident;
SourceLocation IdentLoc;
Decl *Param;
/// DefaultArgTokens - When the parameter's default argument
/// cannot be parsed immediately (because it occurs within the
/// declaration of a member function), it will be stored here as a
/// sequence of tokens to be parsed once the class definition is
/// complete. Non-NULL indicates that there is a default argument.
CachedTokens *DefaultArgTokens;
ParamInfo() {}
ParamInfo(IdentifierInfo *ident, SourceLocation iloc,
Decl *param,
CachedTokens *DefArgTokens = nullptr)
: Ident(ident), IdentLoc(iloc), Param(param),
DefaultArgTokens(DefArgTokens) {}
};
struct TypeAndRange {
ParsedType Ty;
SourceRange Range;
};
struct FunctionTypeInfo : TypeInfoCommon {
/// hasPrototype - This is true if the function had at least one typed
/// parameter. If the function is () or (a,b,c), then it has no prototype,
/// and is treated as a K&R-style function.
unsigned hasPrototype : 1;
/// isVariadic - If this function has a prototype, and if that
/// proto ends with ',...)', this is true. When true, EllipsisLoc
/// contains the location of the ellipsis.
unsigned isVariadic : 1;
/// Can this declaration be a constructor-style initializer?
unsigned isAmbiguous : 1;
/// \brief Whether the ref-qualifier (if any) is an lvalue reference.
/// Otherwise, it's an rvalue reference.
unsigned RefQualifierIsLValueRef : 1;
/// The type qualifiers: const/volatile/restrict.
/// The qualifier bitmask values are the same as in QualType.
unsigned TypeQuals : 3;
/// ExceptionSpecType - An ExceptionSpecificationType value.
unsigned ExceptionSpecType : 4;
/// DeleteParams - If this is true, we need to delete[] Params.
unsigned DeleteParams : 1;
/// HasTrailingReturnType - If this is true, a trailing return type was
/// specified.
unsigned HasTrailingReturnType : 1;
/// The location of the left parenthesis in the source.
unsigned LParenLoc;
/// When isVariadic is true, the location of the ellipsis in the source.
unsigned EllipsisLoc;
/// The location of the right parenthesis in the source.
unsigned RParenLoc;
/// NumParams - This is the number of formal parameters specified by the
/// declarator.
unsigned NumParams;
/// NumExceptions - This is the number of types in the dynamic-exception-
/// decl, if the function has one.
unsigned NumExceptions;
/// \brief The location of the ref-qualifier, if any.
///
/// If this is an invalid location, there is no ref-qualifier.
unsigned RefQualifierLoc;
/// \brief The location of the const-qualifier, if any.
///
/// If this is an invalid location, there is no const-qualifier.
unsigned ConstQualifierLoc;
/// \brief The location of the volatile-qualifier, if any.
///
/// If this is an invalid location, there is no volatile-qualifier.
unsigned VolatileQualifierLoc;
/// \brief The location of the restrict-qualifier, if any.
///
/// If this is an invalid location, there is no restrict-qualifier.
unsigned RestrictQualifierLoc;
/// \brief The location of the 'mutable' qualifer in a lambda-declarator, if
/// any.
unsigned MutableLoc;
/// \brief The beginning location of the exception specification, if any.
unsigned ExceptionSpecLocBeg;
/// \brief The end location of the exception specification, if any.
unsigned ExceptionSpecLocEnd;
/// Params - This is a pointer to a new[]'d array of ParamInfo objects that
/// describe the parameters specified by this function declarator. null if
/// there are no parameters specified.
ParamInfo *Params;
union {
/// \brief Pointer to a new[]'d array of TypeAndRange objects that
/// contain the types in the function's dynamic exception specification
/// and their locations, if there is one.
TypeAndRange *Exceptions;
/// \brief Pointer to the expression in the noexcept-specifier of this
/// function, if it has one.
Expr *NoexceptExpr;
/// \brief Pointer to the cached tokens for an exception-specification
/// that has not yet been parsed.
CachedTokens *ExceptionSpecTokens;
};
/// \brief If HasTrailingReturnType is true, this is the trailing return
/// type specified.
UnionParsedType TrailingReturnType;
/// \brief Reset the parameter list to having zero parameters.
///
/// This is used in various places for error recovery.
void freeParams() {
for (unsigned I = 0; I < NumParams; ++I) {
delete Params[I].DefaultArgTokens;
Params[I].DefaultArgTokens = nullptr;
}
if (DeleteParams) {
delete[] Params;
DeleteParams = false;
}
NumParams = 0;
}
void destroy() {
if (DeleteParams)
delete[] Params;
if (getExceptionSpecType() == EST_Dynamic)
delete[] Exceptions;
else if (getExceptionSpecType() == EST_Unparsed)
delete ExceptionSpecTokens;
}
/// isKNRPrototype - Return true if this is a K&R style identifier list,
/// like "void foo(a,b,c)". In a function definition, this will be followed
/// by the parameter type definitions.
bool isKNRPrototype() const { return !hasPrototype && NumParams != 0; }
SourceLocation getLParenLoc() const {
return SourceLocation::getFromRawEncoding(LParenLoc);
}
SourceLocation getEllipsisLoc() const {
return SourceLocation::getFromRawEncoding(EllipsisLoc);
}
SourceLocation getRParenLoc() const {
return SourceLocation::getFromRawEncoding(RParenLoc);
}
SourceLocation getExceptionSpecLocBeg() const {
return SourceLocation::getFromRawEncoding(ExceptionSpecLocBeg);
}
SourceLocation getExceptionSpecLocEnd() const {
return SourceLocation::getFromRawEncoding(ExceptionSpecLocEnd);
}
SourceRange getExceptionSpecRange() const {
return SourceRange(getExceptionSpecLocBeg(), getExceptionSpecLocEnd());
}
/// \brief Retrieve the location of the ref-qualifier, if any.
SourceLocation getRefQualifierLoc() const {
return SourceLocation::getFromRawEncoding(RefQualifierLoc);
}
/// \brief Retrieve the location of the 'const' qualifier, if any.
SourceLocation getConstQualifierLoc() const {
return SourceLocation::getFromRawEncoding(ConstQualifierLoc);
}
/// \brief Retrieve the location of the 'volatile' qualifier, if any.
SourceLocation getVolatileQualifierLoc() const {
return SourceLocation::getFromRawEncoding(VolatileQualifierLoc);
}
/// \brief Retrieve the location of the 'restrict' qualifier, if any.
SourceLocation getRestrictQualifierLoc() const {
return SourceLocation::getFromRawEncoding(RestrictQualifierLoc);
}
/// \brief Retrieve the location of the 'mutable' qualifier, if any.
SourceLocation getMutableLoc() const {
return SourceLocation::getFromRawEncoding(MutableLoc);
}
/// \brief Determine whether this function declaration contains a
/// ref-qualifier.
bool hasRefQualifier() const { return getRefQualifierLoc().isValid(); }
/// \brief Determine whether this lambda-declarator contains a 'mutable'
/// qualifier.
bool hasMutableQualifier() const { return getMutableLoc().isValid(); }
/// \brief Get the type of exception specification this function has.
ExceptionSpecificationType getExceptionSpecType() const {
return static_cast<ExceptionSpecificationType>(ExceptionSpecType);
}
/// \brief Determine whether this function declarator had a
/// trailing-return-type.
bool hasTrailingReturnType() const { return HasTrailingReturnType; }
/// \brief Get the trailing-return-type for this function declarator.
ParsedType getTrailingReturnType() const { return TrailingReturnType; }
};
struct BlockPointerTypeInfo : TypeInfoCommon {
/// For now, sema will catch these as invalid.
/// The type qualifiers: const/volatile/restrict/_Atomic.
unsigned TypeQuals : 4;
void destroy() {
}
};
struct MemberPointerTypeInfo : TypeInfoCommon {
/// The type qualifiers: const/volatile/restrict/_Atomic.
unsigned TypeQuals : 4;
// CXXScopeSpec has a constructor, so it can't be a direct member.
// So we need some pointer-aligned storage and a bit of trickery.
union {
void *Aligner;
char Mem[sizeof(CXXScopeSpec)];
} ScopeMem;
CXXScopeSpec &Scope() {
return *reinterpret_cast<CXXScopeSpec*>(ScopeMem.Mem);
}
const CXXScopeSpec &Scope() const {
return *reinterpret_cast<const CXXScopeSpec*>(ScopeMem.Mem);
}
void destroy() {
Scope().~CXXScopeSpec();
}
};
struct PipeTypeInfo : TypeInfoCommon {
/// The access writes.
unsigned AccessWrites : 3;
void destroy() {}
};
union {
TypeInfoCommon Common;
PointerTypeInfo Ptr;
ReferenceTypeInfo Ref;
ArrayTypeInfo Arr;
FunctionTypeInfo Fun;
BlockPointerTypeInfo Cls;
MemberPointerTypeInfo Mem;
PipeTypeInfo PipeInfo;
};
void destroy() {
switch (Kind) {
case DeclaratorChunk::Function: return Fun.destroy();
case DeclaratorChunk::Pointer: return Ptr.destroy();
case DeclaratorChunk::BlockPointer: return Cls.destroy();
case DeclaratorChunk::Reference: return Ref.destroy();
case DeclaratorChunk::Array: return Arr.destroy();
case DeclaratorChunk::MemberPointer: return Mem.destroy();
case DeclaratorChunk::Paren: return;
case DeclaratorChunk::Pipe: return PipeInfo.destroy();
}
}
/// \brief If there are attributes applied to this declaratorchunk, return
/// them.
const AttributeList *getAttrs() const {
return Common.AttrList;
}
AttributeList *&getAttrListRef() {
return Common.AttrList;
}
/// \brief Return a DeclaratorChunk for a pointer.
static DeclaratorChunk getPointer(unsigned TypeQuals, SourceLocation Loc,
SourceLocation ConstQualLoc,
SourceLocation VolatileQualLoc,
SourceLocation RestrictQualLoc,
SourceLocation AtomicQualLoc) {
DeclaratorChunk I;
I.Kind = Pointer;
I.Loc = Loc;
I.Ptr.TypeQuals = TypeQuals;
I.Ptr.ConstQualLoc = ConstQualLoc.getRawEncoding();
I.Ptr.VolatileQualLoc = VolatileQualLoc.getRawEncoding();
I.Ptr.RestrictQualLoc = RestrictQualLoc.getRawEncoding();
I.Ptr.AtomicQualLoc = AtomicQualLoc.getRawEncoding();
I.Ptr.AttrList = nullptr;
return I;
}
/// \brief Return a DeclaratorChunk for a reference.
static DeclaratorChunk getReference(unsigned TypeQuals, SourceLocation Loc,
bool lvalue) {
DeclaratorChunk I;
I.Kind = Reference;
I.Loc = Loc;
I.Ref.HasRestrict = (TypeQuals & DeclSpec::TQ_restrict) != 0;
I.Ref.LValueRef = lvalue;
I.Ref.AttrList = nullptr;
return I;
}
/// \brief Return a DeclaratorChunk for an array.
static DeclaratorChunk getArray(unsigned TypeQuals,
bool isStatic, bool isStar, Expr *NumElts,
SourceLocation LBLoc, SourceLocation RBLoc) {
DeclaratorChunk I;
I.Kind = Array;
I.Loc = LBLoc;
I.EndLoc = RBLoc;
I.Arr.AttrList = nullptr;
I.Arr.TypeQuals = TypeQuals;
I.Arr.hasStatic = isStatic;
I.Arr.isStar = isStar;
I.Arr.NumElts = NumElts;
return I;
}
/// DeclaratorChunk::getFunction - Return a DeclaratorChunk for a function.
/// "TheDeclarator" is the declarator that this will be added to.
static DeclaratorChunk getFunction(bool HasProto,
bool IsAmbiguous,
SourceLocation LParenLoc,
ParamInfo *Params, unsigned NumParams,
SourceLocation EllipsisLoc,
SourceLocation RParenLoc,
unsigned TypeQuals,
bool RefQualifierIsLvalueRef,
SourceLocation RefQualifierLoc,
SourceLocation ConstQualifierLoc,
SourceLocation VolatileQualifierLoc,
SourceLocation RestrictQualifierLoc,
SourceLocation MutableLoc,
ExceptionSpecificationType ESpecType,
SourceRange ESpecRange,
ParsedType *Exceptions,
SourceRange *ExceptionRanges,
unsigned NumExceptions,
Expr *NoexceptExpr,
CachedTokens *ExceptionSpecTokens,
SourceLocation LocalRangeBegin,
SourceLocation LocalRangeEnd,
Declarator &TheDeclarator,
TypeResult TrailingReturnType =
TypeResult());
/// \brief Return a DeclaratorChunk for a block.
static DeclaratorChunk getBlockPointer(unsigned TypeQuals,
SourceLocation Loc) {
DeclaratorChunk I;
I.Kind = BlockPointer;
I.Loc = Loc;
I.Cls.TypeQuals = TypeQuals;
I.Cls.AttrList = nullptr;
return I;
}
/// \brief Return a DeclaratorChunk for a block.
static DeclaratorChunk getPipe(unsigned TypeQuals,
SourceLocation Loc) {
DeclaratorChunk I;
I.Kind = Pipe;
I.Loc = Loc;
I.Cls.TypeQuals = TypeQuals;
I.Cls.AttrList = 0;
return I;
}
static DeclaratorChunk getMemberPointer(const CXXScopeSpec &SS,
unsigned TypeQuals,
SourceLocation Loc) {
DeclaratorChunk I;
I.Kind = MemberPointer;
I.Loc = SS.getBeginLoc();
I.EndLoc = Loc;
I.Mem.TypeQuals = TypeQuals;
I.Mem.AttrList = nullptr;
new (I.Mem.ScopeMem.Mem) CXXScopeSpec(SS);
return I;
}
/// \brief Return a DeclaratorChunk for a paren.
static DeclaratorChunk getParen(SourceLocation LParenLoc,
SourceLocation RParenLoc) {
DeclaratorChunk I;
I.Kind = Paren;
I.Loc = LParenLoc;
I.EndLoc = RParenLoc;
I.Common.AttrList = nullptr;
return I;
}
bool isParen() const {
return Kind == Paren;
}
};
/// \brief Described the kind of function definition (if any) provided for
/// a function.
enum FunctionDefinitionKind {
FDK_Declaration,
FDK_Definition,
FDK_Defaulted,
FDK_Deleted
};
/// \brief Information about one declarator, including the parsed type
/// information and the identifier.
///
/// When the declarator is fully formed, this is turned into the appropriate
/// Decl object.
///
/// Declarators come in two types: normal declarators and abstract declarators.
/// Abstract declarators are used when parsing types, and don't have an
/// identifier. Normal declarators do have ID's.
///
/// Instances of this class should be a transient object that lives on the
/// stack, not objects that are allocated in large quantities on the heap.
class Declarator {
public:
enum TheContext {
FileContext, // File scope declaration.
PrototypeContext, // Within a function prototype.
ObjCResultContext, // An ObjC method result type.
ObjCParameterContext,// An ObjC method parameter type.
KNRTypeListContext, // K&R type definition list for formals.
TypeNameContext, // Abstract declarator for types.
MemberContext, // Struct/Union field.
BlockContext, // Declaration within a block in a function.
ForContext, // Declaration within first part of a for loop.
ConditionContext, // Condition declaration in a C++ if/switch/while/for.
TemplateParamContext,// Within a template parameter list.
CXXNewContext, // C++ new-expression.
CXXCatchContext, // C++ catch exception-declaration
ObjCCatchContext, // Objective-C catch exception-declaration
BlockLiteralContext, // Block literal declarator.
LambdaExprContext, // Lambda-expression declarator.
LambdaExprParameterContext, // Lambda-expression parameter declarator.
ConversionIdContext, // C++ conversion-type-id.
TrailingReturnContext, // C++11 trailing-type-specifier.
TemplateTypeArgContext, // Template type argument.
AliasDeclContext, // C++11 alias-declaration.
AliasTemplateContext // C++11 alias-declaration template.
};
private:
const DeclSpec &DS;
CXXScopeSpec SS;
UnqualifiedId Name;
SourceRange Range;
/// \brief Where we are parsing this declarator.
TheContext Context;
/// DeclTypeInfo - This holds each type that the declarator includes as it is
/// parsed. This is pushed from the identifier out, which means that element
/// #0 will be the most closely bound to the identifier, and
/// DeclTypeInfo.back() will be the least closely bound.
SmallVector<DeclaratorChunk, 8> DeclTypeInfo;
/// InvalidType - Set by Sema::GetTypeForDeclarator().
bool InvalidType : 1;
/// GroupingParens - Set by Parser::ParseParenDeclarator().
bool GroupingParens : 1;
/// FunctionDefinition - Is this Declarator for a function or member
/// definition and, if so, what kind?
///
/// Actually a FunctionDefinitionKind.
unsigned FunctionDefinition : 2;
/// \brief Is this Declarator a redeclaration?
bool Redeclaration : 1;
/// Attrs - Attributes.
ParsedAttributes Attrs;
/// \brief The asm label, if specified.
Expr *AsmLabel;
/// InlineParams - This is a local array used for the first function decl
/// chunk to avoid going to the heap for the common case when we have one
/// function chunk in the declarator.
DeclaratorChunk::ParamInfo InlineParams[16];
bool InlineParamsUsed;
/// \brief true if the declaration is preceded by \c __extension__.
unsigned Extension : 1;
/// Indicates whether this is an Objective-C instance variable.
unsigned ObjCIvar : 1;
/// Indicates whether this is an Objective-C 'weak' property.
unsigned ObjCWeakProperty : 1;
/// \brief If this is the second or subsequent declarator in this declaration,
/// the location of the comma before this declarator.
SourceLocation CommaLoc;
/// \brief If provided, the source location of the ellipsis used to describe
/// this declarator as a parameter pack.
SourceLocation EllipsisLoc;
friend struct DeclaratorChunk;
public:
Declarator(const DeclSpec &ds, TheContext C)
: DS(ds), Range(ds.getSourceRange()), Context(C),
InvalidType(DS.getTypeSpecType() == DeclSpec::TST_error),
GroupingParens(false), FunctionDefinition(FDK_Declaration),
Redeclaration(false),
Attrs(ds.getAttributePool().getFactory()), AsmLabel(nullptr),
InlineParamsUsed(false), Extension(false), ObjCIvar(false),
ObjCWeakProperty(false) {
}
~Declarator() {
clear();
}
/// getDeclSpec - Return the declaration-specifier that this declarator was
/// declared with.
const DeclSpec &getDeclSpec() const { return DS; }
/// getMutableDeclSpec - Return a non-const version of the DeclSpec. This
/// should be used with extreme care: declspecs can often be shared between
/// multiple declarators, so mutating the DeclSpec affects all of the
/// Declarators. This should only be done when the declspec is known to not
/// be shared or when in error recovery etc.
DeclSpec &getMutableDeclSpec() { return const_cast<DeclSpec &>(DS); }
AttributePool &getAttributePool() const {
return Attrs.getPool();
}
/// getCXXScopeSpec - Return the C++ scope specifier (global scope or
/// nested-name-specifier) that is part of the declarator-id.
const CXXScopeSpec &getCXXScopeSpec() const { return SS; }
CXXScopeSpec &getCXXScopeSpec() { return SS; }
/// \brief Retrieve the name specified by this declarator.
UnqualifiedId &getName() { return Name; }
TheContext getContext() const { return Context; }
bool isPrototypeContext() const {
return (Context == PrototypeContext ||
Context == ObjCParameterContext ||
Context == ObjCResultContext ||
Context == LambdaExprParameterContext);
}
/// \brief Get the source range that spans this declarator.
SourceRange getSourceRange() const LLVM_READONLY { return Range; }
SourceLocation getLocStart() const LLVM_READONLY { return Range.getBegin(); }
SourceLocation getLocEnd() const LLVM_READONLY { return Range.getEnd(); }
void SetSourceRange(SourceRange R) { Range = R; }
/// SetRangeBegin - Set the start of the source range to Loc, unless it's
/// invalid.
void SetRangeBegin(SourceLocation Loc) {
if (!Loc.isInvalid())
Range.setBegin(Loc);
}
/// SetRangeEnd - Set the end of the source range to Loc, unless it's invalid.
void SetRangeEnd(SourceLocation Loc) {
if (!Loc.isInvalid())
Range.setEnd(Loc);
}
/// ExtendWithDeclSpec - Extend the declarator source range to include the
/// given declspec, unless its location is invalid. Adopts the range start if
/// the current range start is invalid.
void ExtendWithDeclSpec(const DeclSpec &DS) {
SourceRange SR = DS.getSourceRange();
if (Range.getBegin().isInvalid())
Range.setBegin(SR.getBegin());
if (!SR.getEnd().isInvalid())
Range.setEnd(SR.getEnd());
}
/// \brief Reset the contents of this Declarator.
void clear() {
SS.clear();
Name.clear();
Range = DS.getSourceRange();
for (unsigned i = 0, e = DeclTypeInfo.size(); i != e; ++i)
DeclTypeInfo[i].destroy();
DeclTypeInfo.clear();
Attrs.clear();
AsmLabel = nullptr;
InlineParamsUsed = false;
ObjCIvar = false;
ObjCWeakProperty = false;
CommaLoc = SourceLocation();
EllipsisLoc = SourceLocation();
}
/// mayOmitIdentifier - Return true if the identifier is either optional or
/// not allowed. This is true for typenames, prototypes, and template
/// parameter lists.
bool mayOmitIdentifier() const {
switch (Context) {
case FileContext:
case KNRTypeListContext:
case MemberContext:
case BlockContext:
case ForContext:
case ConditionContext:
return false;
case TypeNameContext:
case AliasDeclContext:
case AliasTemplateContext:
case PrototypeContext:
case LambdaExprParameterContext:
case ObjCParameterContext:
case ObjCResultContext:
case TemplateParamContext:
case CXXNewContext:
case CXXCatchContext:
case ObjCCatchContext:
case BlockLiteralContext:
case LambdaExprContext:
case ConversionIdContext:
case TemplateTypeArgContext:
case TrailingReturnContext:
return true;
}
llvm_unreachable("unknown context kind!");
}
/// mayHaveIdentifier - Return true if the identifier is either optional or
/// required. This is true for normal declarators and prototypes, but not
/// typenames.
bool mayHaveIdentifier() const {
switch (Context) {
case FileContext:
case KNRTypeListContext:
case MemberContext:
case BlockContext:
case ForContext:
case ConditionContext:
case PrototypeContext:
case LambdaExprParameterContext:
case TemplateParamContext:
case CXXCatchContext:
case ObjCCatchContext:
return true;
case TypeNameContext:
case CXXNewContext:
case AliasDeclContext:
case AliasTemplateContext:
case ObjCParameterContext:
case ObjCResultContext:
case BlockLiteralContext:
case LambdaExprContext:
case ConversionIdContext:
case TemplateTypeArgContext:
case TrailingReturnContext:
return false;
}
llvm_unreachable("unknown context kind!");
}
/// diagnoseIdentifier - Return true if the identifier is prohibited and
/// should be diagnosed (because it cannot be anything else).
bool diagnoseIdentifier() const {
switch (Context) {
case FileContext:
case KNRTypeListContext:
case MemberContext:
case BlockContext:
case ForContext:
case ConditionContext:
case PrototypeContext:
case LambdaExprParameterContext:
case TemplateParamContext:
case CXXCatchContext:
case ObjCCatchContext:
case TypeNameContext:
case ConversionIdContext:
case ObjCParameterContext:
case ObjCResultContext:
case BlockLiteralContext:
case CXXNewContext:
case LambdaExprContext:
return false;
case AliasDeclContext:
case AliasTemplateContext:
case TemplateTypeArgContext:
case TrailingReturnContext:
return true;
}
llvm_unreachable("unknown context kind!");
}
/// mayBeFollowedByCXXDirectInit - Return true if the declarator can be
/// followed by a C++ direct initializer, e.g. "int x(1);".
bool mayBeFollowedByCXXDirectInit() const {
if (hasGroupingParens()) return false;
if (getDeclSpec().getStorageClassSpec() == DeclSpec::SCS_typedef)
return false;
if (getDeclSpec().getStorageClassSpec() == DeclSpec::SCS_extern &&
Context != FileContext)
return false;
// Special names can't have direct initializers.
if (Name.getKind() != UnqualifiedId::IK_Identifier)
return false;
switch (Context) {
case FileContext:
case BlockContext:
case ForContext:
return true;
case ConditionContext:
// This may not be followed by a direct initializer, but it can't be a
// function declaration either, and we'd prefer to perform a tentative
// parse in order to produce the right diagnostic.
return true;
case KNRTypeListContext:
case MemberContext:
case PrototypeContext:
case LambdaExprParameterContext:
case ObjCParameterContext:
case ObjCResultContext:
case TemplateParamContext:
case CXXCatchContext:
case ObjCCatchContext:
case TypeNameContext:
case CXXNewContext:
case AliasDeclContext:
case AliasTemplateContext:
case BlockLiteralContext:
case LambdaExprContext:
case ConversionIdContext:
case TemplateTypeArgContext:
case TrailingReturnContext:
return false;
}
llvm_unreachable("unknown context kind!");
}
/// isPastIdentifier - Return true if we have parsed beyond the point where
/// the
bool isPastIdentifier() const { return Name.isValid(); }
/// hasName - Whether this declarator has a name, which might be an
/// identifier (accessible via getIdentifier()) or some kind of
/// special C++ name (constructor, destructor, etc.).
bool hasName() const {
return Name.getKind() != UnqualifiedId::IK_Identifier || Name.Identifier;
}
IdentifierInfo *getIdentifier() const {
if (Name.getKind() == UnqualifiedId::IK_Identifier)
return Name.Identifier;
return nullptr;
}
SourceLocation getIdentifierLoc() const { return Name.StartLocation; }
/// \brief Set the name of this declarator to be the given identifier.
void SetIdentifier(IdentifierInfo *Id, SourceLocation IdLoc) {
Name.setIdentifier(Id, IdLoc);
}
/// AddTypeInfo - Add a chunk to this declarator. Also extend the range to
/// EndLoc, which should be the last token of the chunk.
void AddTypeInfo(const DeclaratorChunk &TI,
ParsedAttributes &attrs,
SourceLocation EndLoc) {
DeclTypeInfo.push_back(TI);
DeclTypeInfo.back().getAttrListRef() = attrs.getList();
getAttributePool().takeAllFrom(attrs.getPool());
if (!EndLoc.isInvalid())
SetRangeEnd(EndLoc);
}
/// \brief Add a new innermost chunk to this declarator.
void AddInnermostTypeInfo(const DeclaratorChunk &TI) {
DeclTypeInfo.insert(DeclTypeInfo.begin(), TI);
}
/// \brief Return the number of types applied to this declarator.
unsigned getNumTypeObjects() const { return DeclTypeInfo.size(); }
/// Return the specified TypeInfo from this declarator. TypeInfo #0 is
/// closest to the identifier.
const DeclaratorChunk &getTypeObject(unsigned i) const {
assert(i < DeclTypeInfo.size() && "Invalid type chunk");
return DeclTypeInfo[i];
}
DeclaratorChunk &getTypeObject(unsigned i) {
assert(i < DeclTypeInfo.size() && "Invalid type chunk");
return DeclTypeInfo[i];
}
typedef SmallVectorImpl<DeclaratorChunk>::const_iterator type_object_iterator;
typedef llvm::iterator_range<type_object_iterator> type_object_range;
/// Returns the range of type objects, from the identifier outwards.
type_object_range type_objects() const {
return type_object_range(DeclTypeInfo.begin(), DeclTypeInfo.end());
}
void DropFirstTypeObject() {
assert(!DeclTypeInfo.empty() && "No type chunks to drop.");
DeclTypeInfo.front().destroy();
DeclTypeInfo.erase(DeclTypeInfo.begin());
}
/// Return the innermost (closest to the declarator) chunk of this
/// declarator that is not a parens chunk, or null if there are no
/// non-parens chunks.
const DeclaratorChunk *getInnermostNonParenChunk() const {
for (unsigned i = 0, i_end = DeclTypeInfo.size(); i < i_end; ++i) {
if (!DeclTypeInfo[i].isParen())
return &DeclTypeInfo[i];
}
return nullptr;
}
/// Return the outermost (furthest from the declarator) chunk of
/// this declarator that is not a parens chunk, or null if there are
/// no non-parens chunks.
const DeclaratorChunk *getOutermostNonParenChunk() const {
for (unsigned i = DeclTypeInfo.size(), i_end = 0; i != i_end; --i) {
if (!DeclTypeInfo[i-1].isParen())
return &DeclTypeInfo[i-1];
}
return nullptr;
}
/// isArrayOfUnknownBound - This method returns true if the declarator
/// is a declarator for an array of unknown bound (looking through
/// parentheses).
bool isArrayOfUnknownBound() const {
const DeclaratorChunk *chunk = getInnermostNonParenChunk();
return (chunk && chunk->Kind == DeclaratorChunk::Array &&
!chunk->Arr.NumElts);
}
/// isFunctionDeclarator - This method returns true if the declarator
/// is a function declarator (looking through parentheses).
/// If true is returned, then the reference type parameter idx is
/// assigned with the index of the declaration chunk.
bool isFunctionDeclarator(unsigned& idx) const {
for (unsigned i = 0, i_end = DeclTypeInfo.size(); i < i_end; ++i) {
switch (DeclTypeInfo[i].Kind) {
case DeclaratorChunk::Function:
idx = i;
return true;
case DeclaratorChunk::Paren:
continue;
case DeclaratorChunk::Pointer:
case DeclaratorChunk::Reference:
case DeclaratorChunk::Array:
case DeclaratorChunk::BlockPointer:
case DeclaratorChunk::MemberPointer:
case DeclaratorChunk::Pipe:
return false;
}
llvm_unreachable("Invalid type chunk");
}
return false;
}
/// isFunctionDeclarator - Once this declarator is fully parsed and formed,
/// this method returns true if the identifier is a function declarator
/// (looking through parentheses).
bool isFunctionDeclarator() const {
unsigned index;
return isFunctionDeclarator(index);
}
/// getFunctionTypeInfo - Retrieves the function type info object
/// (looking through parentheses).
DeclaratorChunk::FunctionTypeInfo &getFunctionTypeInfo() {
assert(isFunctionDeclarator() && "Not a function declarator!");
unsigned index = 0;
isFunctionDeclarator(index);
return DeclTypeInfo[index].Fun;
}
/// getFunctionTypeInfo - Retrieves the function type info object
/// (looking through parentheses).
const DeclaratorChunk::FunctionTypeInfo &getFunctionTypeInfo() const {
return const_cast<Declarator*>(this)->getFunctionTypeInfo();
}
/// \brief Determine whether the declaration that will be produced from
/// this declaration will be a function.
///
/// A declaration can declare a function even if the declarator itself
/// isn't a function declarator, if the type specifier refers to a function
/// type. This routine checks for both cases.
bool isDeclarationOfFunction() const;
/// \brief Return true if this declaration appears in a context where a
/// function declarator would be a function declaration.
bool isFunctionDeclarationContext() const {
if (getDeclSpec().getStorageClassSpec() == DeclSpec::SCS_typedef)
return false;
switch (Context) {
case FileContext:
case MemberContext:
case BlockContext:
return true;
case ForContext:
case ConditionContext:
case KNRTypeListContext:
case TypeNameContext:
case AliasDeclContext:
case AliasTemplateContext:
case PrototypeContext:
case LambdaExprParameterContext:
case ObjCParameterContext:
case ObjCResultContext:
case TemplateParamContext:
case CXXNewContext:
case CXXCatchContext:
case ObjCCatchContext:
case BlockLiteralContext:
case LambdaExprContext:
case ConversionIdContext:
case TemplateTypeArgContext:
case TrailingReturnContext:
return false;
}
llvm_unreachable("unknown context kind!");
}
/// \brief Return true if a function declarator at this position would be a
/// function declaration.
bool isFunctionDeclaratorAFunctionDeclaration() const {
if (!isFunctionDeclarationContext())
return false;
for (unsigned I = 0, N = getNumTypeObjects(); I != N; ++I)
if (getTypeObject(I).Kind != DeclaratorChunk::Paren)
return false;
return true;
}
/// takeAttributes - Takes attributes from the given parsed-attributes
/// set and add them to this declarator.
///
/// These examples both add 3 attributes to "var":
/// short int var __attribute__((aligned(16),common,deprecated));
/// short int x, __attribute__((aligned(16)) var
/// __attribute__((common,deprecated));
///
/// Also extends the range of the declarator.
void takeAttributes(ParsedAttributes &attrs, SourceLocation lastLoc) {
Attrs.takeAllFrom(attrs);
if (!lastLoc.isInvalid())
SetRangeEnd(lastLoc);
}
const AttributeList *getAttributes() const { return Attrs.getList(); }
AttributeList *getAttributes() { return Attrs.getList(); }
AttributeList *&getAttrListRef() { return Attrs.getListRef(); }
/// hasAttributes - do we contain any attributes?
bool hasAttributes() const {
if (getAttributes() || getDeclSpec().hasAttributes()) return true;
for (unsigned i = 0, e = getNumTypeObjects(); i != e; ++i)
if (getTypeObject(i).getAttrs())
return true;
return false;
}
/// \brief Return a source range list of C++11 attributes associated
/// with the declarator.
void getCXX11AttributeRanges(SmallVectorImpl<SourceRange> &Ranges) {
AttributeList *AttrList = Attrs.getList();
while (AttrList) {
if (AttrList->isCXX11Attribute())
Ranges.push_back(AttrList->getRange());
AttrList = AttrList->getNext();
}
}
void setAsmLabel(Expr *E) { AsmLabel = E; }
Expr *getAsmLabel() const { return AsmLabel; }
void setExtension(bool Val = true) { Extension = Val; }
bool getExtension() const { return Extension; }
void setObjCIvar(bool Val = true) { ObjCIvar = Val; }
bool isObjCIvar() const { return ObjCIvar; }
void setObjCWeakProperty(bool Val = true) { ObjCWeakProperty = Val; }
bool isObjCWeakProperty() const { return ObjCWeakProperty; }
void setInvalidType(bool Val = true) { InvalidType = Val; }
bool isInvalidType() const {
return InvalidType || DS.getTypeSpecType() == DeclSpec::TST_error;
}
void setGroupingParens(bool flag) { GroupingParens = flag; }
bool hasGroupingParens() const { return GroupingParens; }
bool isFirstDeclarator() const { return !CommaLoc.isValid(); }
SourceLocation getCommaLoc() const { return CommaLoc; }
void setCommaLoc(SourceLocation CL) { CommaLoc = CL; }
bool hasEllipsis() const { return EllipsisLoc.isValid(); }
SourceLocation getEllipsisLoc() const { return EllipsisLoc; }
void setEllipsisLoc(SourceLocation EL) { EllipsisLoc = EL; }
void setFunctionDefinitionKind(FunctionDefinitionKind Val) {
FunctionDefinition = Val;
}
bool isFunctionDefinition() const {
return getFunctionDefinitionKind() != FDK_Declaration;
}
FunctionDefinitionKind getFunctionDefinitionKind() const {
return (FunctionDefinitionKind)FunctionDefinition;
}
/// Returns true if this declares a real member and not a friend.
bool isFirstDeclarationOfMember() {
return getContext() == MemberContext && !getDeclSpec().isFriendSpecified();
}
/// Returns true if this declares a static member. This cannot be called on a
/// declarator outside of a MemberContext because we won't know until
/// redeclaration time if the decl is static.
bool isStaticMember();
/// Returns true if this declares a constructor or a destructor.
bool isCtorOrDtor();
void setRedeclaration(bool Val) { Redeclaration = Val; }
bool isRedeclaration() const { return Redeclaration; }
};
/// \brief This little struct is used to capture information about
/// structure field declarators, which is basically just a bitfield size.
struct FieldDeclarator {
Declarator D;
Expr *BitfieldSize;
explicit FieldDeclarator(const DeclSpec &DS)
: D(DS, Declarator::MemberContext), BitfieldSize(nullptr) { }
};
/// \brief Represents a C++11 virt-specifier-seq.
class VirtSpecifiers {
public:
enum Specifier {
VS_None = 0,
VS_Override = 1,
VS_Final = 2,
VS_Sealed = 4
};
VirtSpecifiers() : Specifiers(0), LastSpecifier(VS_None) { }
bool SetSpecifier(Specifier VS, SourceLocation Loc,
const char *&PrevSpec);
bool isUnset() const { return Specifiers == 0; }
bool isOverrideSpecified() const { return Specifiers & VS_Override; }
SourceLocation getOverrideLoc() const { return VS_overrideLoc; }
bool isFinalSpecified() const { return Specifiers & (VS_Final | VS_Sealed); }
bool isFinalSpelledSealed() const { return Specifiers & VS_Sealed; }
SourceLocation getFinalLoc() const { return VS_finalLoc; }
void clear() { Specifiers = 0; }
static const char *getSpecifierName(Specifier VS);
SourceLocation getFirstLocation() const { return FirstLocation; }
SourceLocation getLastLocation() const { return LastLocation; }
Specifier getLastSpecifier() const { return LastSpecifier; }
private:
unsigned Specifiers;
Specifier LastSpecifier;
SourceLocation VS_overrideLoc, VS_finalLoc;
SourceLocation FirstLocation;
SourceLocation LastLocation;
};
enum class LambdaCaptureInitKind {
NoInit, //!< [a]
CopyInit, //!< [a = b], [a = {b}]
DirectInit, //!< [a(b)]
ListInit //!< [a{b}]
};
/// \brief Represents a complete lambda introducer.
struct LambdaIntroducer {
/// \brief An individual capture in a lambda introducer.
struct LambdaCapture {
LambdaCaptureKind Kind;
SourceLocation Loc;
IdentifierInfo *Id;
SourceLocation EllipsisLoc;
LambdaCaptureInitKind InitKind;
ExprResult Init;
ParsedType InitCaptureType;
LambdaCapture(LambdaCaptureKind Kind, SourceLocation Loc,
IdentifierInfo *Id, SourceLocation EllipsisLoc,
LambdaCaptureInitKind InitKind, ExprResult Init,
ParsedType InitCaptureType)
: Kind(Kind), Loc(Loc), Id(Id), EllipsisLoc(EllipsisLoc),
InitKind(InitKind), Init(Init), InitCaptureType(InitCaptureType) {}
};
SourceRange Range;
SourceLocation DefaultLoc;
LambdaCaptureDefault Default;
SmallVector<LambdaCapture, 4> Captures;
LambdaIntroducer()
: Default(LCD_None) {}
/// \brief Append a capture in a lambda introducer.
void addCapture(LambdaCaptureKind Kind,
SourceLocation Loc,
IdentifierInfo* Id,
SourceLocation EllipsisLoc,
LambdaCaptureInitKind InitKind,
ExprResult Init,
ParsedType InitCaptureType) {
Captures.push_back(LambdaCapture(Kind, Loc, Id, EllipsisLoc, InitKind, Init,
InitCaptureType));
}
};
} // end namespace clang
#endif
| {
"content_hash": "672d47da21127d28b6784395abbf5fb0",
"timestamp": "",
"source": "github",
"line_count": 2343,
"max_line_length": 81,
"avg_line_length": 35.87836107554418,
"alnum_prop": 0.6782532148507667,
"repo_name": "mirams/opencor",
"id": "67d7b5c3fb479b48dc9ccc5bfaeeb6c5f2487218",
"size": "84063",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "src/plugins/thirdParty/LLVM/tools/clang/include/clang/Sema/DeclSpec.h",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "Batchfile",
"bytes": "2211"
},
{
"name": "C",
"bytes": "7562304"
},
{
"name": "C++",
"bytes": "114016189"
},
{
"name": "CMake",
"bytes": "216057"
},
{
"name": "HTML",
"bytes": "9433"
},
{
"name": "NSIS",
"bytes": "4599"
},
{
"name": "Objective-C",
"bytes": "282234"
},
{
"name": "PAWN",
"bytes": "136"
},
{
"name": "PHP",
"bytes": "154028"
},
{
"name": "POV-Ray SDL",
"bytes": "32617292"
},
{
"name": "Shell",
"bytes": "2656"
},
{
"name": "SourcePawn",
"bytes": "1544"
},
{
"name": "Visual Basic",
"bytes": "332"
},
{
"name": "XSLT",
"bytes": "59631"
}
],
"symlink_target": ""
} |
"""Run AFL repeatedly with externally supplied generated packet from STDIN."""
import logging
import sys
import afl
from ryu.controller import dpset
from faucet import faucet
from faucet import faucet_experimental_api
import fake_packet
ROUNDS = 1
logging.disable(logging.CRITICAL)
def main():
"""Run AFL repeatedly with externally supplied generated packet from STDIN."""
application = faucet.Faucet(
dpset=dpset.DPSet(),
faucet_experimental_api=faucet_experimental_api.FaucetExperimentalAPI())
application.start()
# make sure dps are running
if application.valves_manager is not None:
for valve in list(application.valves_manager.valves.values()):
state = valve.dp.dyn_finalized
valve.dp.dyn_finalized = False
valve.dp.running = True
valve.dp.dyn_finalized = state
while afl.loop(ROUNDS): # pylint: disable=c-extension-no-member
# receive input from afl
rcv = sys.stdin.read()
data = None
try:
data = bytearray.fromhex(rcv) # pytype: disable=missing-parameter,wrong-arg-types
except (ValueError, TypeError):
continue
# create fake packet
_dp = fake_packet.Datapath(1)
msg = fake_packet.Message(datapath=_dp, cookie=15243729, port=1, data=data, in_port=1)
pkt = fake_packet.RyuEvent(msg)
# send fake packet to faucet
application.packet_in_handler(pkt)
if __name__ == "__main__":
main()
| {
"content_hash": "7b3396889d8e82b1750cc28989093c2e",
"timestamp": "",
"source": "github",
"line_count": 50,
"max_line_length": 94,
"avg_line_length": 30.22,
"alnum_prop": 0.6591661151555261,
"repo_name": "gizmoguy/faucet",
"id": "703e72de0f185b60d81e08cc9fa3d1c8fce71a12",
"size": "1535",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "tests/fuzzer/fuzz_packet.py",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "Makefile",
"bytes": "2538"
},
{
"name": "Python",
"bytes": "1779977"
},
{
"name": "Shell",
"bytes": "18018"
}
],
"symlink_target": ""
} |
/*
Created by TheRacingLion (https://github.com/TheRacingLion) [ 03 / 03 / 2017 ]
-*Read LICENSE to know more about permissions*-
Config Validator. Checks if the config is valid before letting the bot start.
*/
const moment = require('moment')
const chalk = require('chalk')
const status = {
online: `${chalk.green('"online"')}`,
idle: `${chalk.yellow('"idle"')}`,
dnd: `${chalk.red('"dnd"')} (Do Not Disturb)`,
invisible: '"invisible"'
}
function Err (key, type = '') {
if (type === 'bol') type = '(Must be either true or false)'
console.error(`\n[${chalk.cyan(moment().format('H:mm:ss'))}]${chalk.bgRed.bold(' Config Error ')} Invalid ${key}. ${type}.\n`)
process.exit()
}
module.exports.check = function (config, log) {
log.log('Checking Config File...', 'Config', 'bgCyan', true)
if (config.token === '' || typeof config.token !== 'string') {
Err('Token')
} else if (!(/^\d{17,18}/.test(config.ownerID)) || typeof config.ownerID !== 'string') {
Err('ownerID', 'Must be a string of your discord ID')
} else if (config.prefix === '' || typeof config.prefix !== 'string') {
Err('Prefix')
} else if (typeof config.rotatePlayingGame !== 'boolean') {
Err('rotatePlayingGame', 'bol')
} else if (config.rotatePlayingGame && (isNaN(config.rotatePlayingGameTime) || config.rotatePlayingGameTime <= 5000)) {
Err('rotatePlayingGameTime', 'Must be a integer number bigger than 5000 (5 seconds).')
} else if (typeof config.rotatePlayingGameInStreamingStatus !== 'boolean') {
Err('rotatePlayingGameInStreamingStatus', 'bol')
} else if (typeof config.rotateAvatarImage !== 'boolean') {
Err('rotateAvatarImage', 'bol')
} else if (config.rotateAvatarImage && (isNaN(config.rotateAvatarImageTime) || config.rotateAvatarImageTime < 600000)) {
Err('rotateAvatarImageTime', 'Must be a integer number bigger than 300000 (5 minutes).')
} else if (typeof config.defaultStatus !== 'string' || Object.keys(status).indexOf(config.defaultStatus.toLowerCase()) < 0) {
Err(`defaultStatus. Must be either:\n${Object.values(status).join(', ')}`)
} else if (typeof config.mentionNotificator !== 'object') {
Err('mentionNotificator', 'Must be an object with two options:\n\n"mentionNotificator": {\n "inConsole": true,\n "inNotificationChannel": true\n}')
} else if (typeof config.mentionNotificator.inConsole !== 'boolean') {
Err('mentionNotificator -> "inConsole"', 'bol')
} else if (typeof config.mentionNotificator.inNotificationChannel !== 'boolean') {
Err('mentionNotificator -> "inNotificationChannel"', 'bol')
} else if (typeof config.mentionNotificator.logBlockedUsers !== 'boolean') {
Err('mentionNotificator -> "logBlockedUsers"', 'bol')
} else if (!Array.isArray(config.mentionNotificator.ignoredServers)) {
Err('mentionNotificator -> "ignoredServers"', 'Must be an array')
} else if (typeof config.keywordNotificator !== 'object') {
Err('keywordNotificator', 'Must be an object with two options:\n\n"keywordNotificator": {\n "inConsole": true,\n "inNotificationChannel": true\n}')
} else if (typeof config.keywordNotificator.inConsole !== 'boolean') {
Err('keywordNotificator -> "inConsole"', 'bol')
} else if (typeof config.keywordNotificator.inNotificationChannel !== 'boolean') {
Err('keywordNotificator -> "inNotificationChannel"', 'bol')
} else if (typeof config.keywordNotificator.logBlockedUsers !== 'boolean') {
Err('keywordNotificator -> "logBlockedUsers"', 'bol')
} else if (typeof config.keywordNotificator.caseInsensitive !== 'boolean') {
Err('keywordNotificator -> "caseInsensitive"', 'bol')
} else if (!Array.isArray(config.keywordNotificator.ignoredServers)) {
Err('keywordNotificator -> "ignoredServers"', 'Must be an array')
} else if (typeof config.eventNotificator !== 'object') {
Err('eventNotificator', 'Must be an object with two options:\n\n"eventNotificator": {\n "inConsole": true,\n "inNotificationChannel": true\n}')
} else if (typeof config.eventNotificator.inConsole !== 'boolean') {
Err('eventNotificator -> "inConsole"', 'bol')
} else if (typeof config.eventNotificator.inNotificationChannel !== 'boolean') {
Err('eventNotificator -> "inNotificationChannel"', 'bol')
} else if ((config.mentionNotificator.inNotificationChannel || config.eventNotificator.inNotificationChannel || config.keywordNotificator.inNotificationChannel) && !(/^\d{17,18}/.test(config.notificationChannelID))) {
Err('notificationChannelID', 'Must be a channel ID from a server you are in.')
} else if (!/#?([\da-fA-F]{2})([\da-fA-F]{2})([\da-fA-F]{2})/i.test(config.defaultEmbedColor)) {
Err('defaultEmbedColor', 'Must be a valid hex color code.')
} else if (typeof config.deleteCommandMessages !== 'boolean') {
Err('deleteCommandMessages', 'bol')
} else if (config.deleteCommandMessages && isNaN(config.deleteCommandMessagesTime)) {
Err('deleteCommandMessagesTime', 'Must be a integer number.')
} else {
log.log('Config is valid. Starting Bot...', 'Config', 'bgCyan', true)
}
}
| {
"content_hash": "0343f00d05a7f4307016379dbcb9d181",
"timestamp": "",
"source": "github",
"line_count": 81,
"max_line_length": 219,
"avg_line_length": 62.54320987654321,
"alnum_prop": 0.688511646269246,
"repo_name": "NovalFuzzy/Updated-Selfbot",
"id": "096babec81c612796a528732ee27c1d128e01d1c",
"size": "5066",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "src/utils/ConfigValidator.js",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "Batchfile",
"bytes": "1004"
},
{
"name": "JavaScript",
"bytes": "38226"
}
],
"symlink_target": ""
} |
<?php
// Copyright 2017 DAIMTO ([Linda Lawton](https://twitter.com/LindaLawtonDK)) : [www.daimto.com](http://www.daimto.com/)
//
// Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
// the License. You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
// an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
// specific language governing permissions and limitations under the License.
//------------------------------------------------------------------------------
// <auto-generated>
// This code was generated by DAIMTO-Google-apis-Sample-generator 1.0.0
// Template File Name: methodTemplate.tt
// Build date: 2017-10-08
// PHP generator version: 1.0.0
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
// </auto-generated>
//------------------------------------------------------------------------------
// About
//
// Unofficial sample for the plus v1 API for PHP.
// This sample is designed to be used with the Google PHP client library. (https://github.com/google/google-api-php-client)
//
// API Description: Builds on top of the Google+ platform.
// API Documentation Link https://developers.google.com/+/api/
//
// Discovery Doc https://www.googleapis.com/discovery/v1/apis/plus/v1/rest
//
//------------------------------------------------------------------------------
// Installation
//
// The preferred method is via https://getcomposer.org. Follow the installation instructions https://getcomposer.org/doc/00-intro.md
// if you do not already have composer installed.
//
// Once composer is installed, execute the following command in your project root to install this library:
//
// composer require google/apiclient:^2.0
//
//------------------------------------------------------------------------------
// Load the Google API PHP Client Library.
require_once __DIR__ . '/vendor/autoload.php';
session_start();
/***************************************************
* Include this line for service account authencation. Note: Not all APIs support service accounts.
//require_once __DIR__ . '/ServiceAccount.php';
* Include the following four lines Oauth2 authencation.
* require_once __DIR__ . '/Oauth2Authentication.php';
* $_SESSION['mainScript'] = basename($_SERVER['PHP_SELF']); // Oauth2callback.php will return here.
* $client = getGoogleClient();
* $service = new Google_Service_Plus($client);
****************************************************/
// Option paramaters can be set as needed.
$optParams = array(
'fields' => '*'
);
// Single Request.
$results = peopleGetExample($service, $userId, $optParams);
/**
* Get a person's profile. If your app uses scope https://www.googleapis.com/auth/plus.login, this method is guaranteed to return ageRange and language.
* @service Authenticated Plus service.
* @optParams Optional paramaters are not required by a request.
* @userId The ID of the person to get the profile for. The special value "me" can be used to indicate the authenticated user.
* @return Person
*/
function peopleGetExample($service, $userId, $optParams)
{
try
{
// Parameter validation.
if ($service == null)
throw new Exception("service is required.");
if ($optParams == null)
throw new Exception("optParams is required.");
if (userId == null)
throw new Exception("userId is required.");
// Make the request and return the results.
return $service->people->GetPeople($userId, $optParams);
}
catch (Exception $e)
{
print "An error occurred: " . $e->getMessage();
}
}
?>
| {
"content_hash": "cfdda4acc9822bf21d600c6da8a4ca0b",
"timestamp": "",
"source": "github",
"line_count": 92,
"max_line_length": 151,
"avg_line_length": 41.73913043478261,
"alnum_prop": 0.63515625,
"repo_name": "LindaLawton/Google-APIs-PHP-Samples",
"id": "84ed29bb2a120f05bec4d86d141ca5e1bcbe8268",
"size": "3842",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "Samples/Google+ API/v1/PeopleGetSample.php",
"mode": "33188",
"license": "apache-2.0",
"language": [],
"symlink_target": ""
} |
#include <linux/skbuff.h>
#include <linux/string.h>
#include <linux/module.h>
#include <asm/byteorder.h>
#include <net/irda/irda.h>
#include <net/irda/wrapper.h>
#include <net/irda/crc.h>
#include <net/irda/irlap.h>
#include <net/irda/irlap_frame.h>
#include <net/irda/irda_device.h>
/************************** FRAME WRAPPING **************************/
/*
* Unwrap and unstuff SIR frames
*
* Note : at FIR and MIR, HDLC framing is used and usually handled
* by the controller, so we come here only for SIR... Jean II
*/
/*
* Function stuff_byte (byte, buf)
*
* Byte stuff one single byte and put the result in buffer pointed to by
* buf. The buffer must at all times be able to have two bytes inserted.
*
* This is in a tight loop, better inline it, so need to be prior to callers.
* (2000 bytes on P6 200MHz, non-inlined ~370us, inline ~170us) - Jean II
*/
static inline int stuff_byte(__u8 byte, __u8 *buf)
{
switch (byte) {
case BOF: /* FALLTHROUGH */
case EOF: /* FALLTHROUGH */
case CE:
/* Insert transparently coded */
buf[0] = CE; /* Send link escape */
buf[1] = byte^IRDA_TRANS; /* Complement bit 5 */
return 2;
/* break; */
default:
/* Non-special value, no transparency required */
buf[0] = byte;
return 1;
/* break; */
}
}
/*
* Function async_wrap (skb, *tx_buff, buffsize)
*
* Makes a new buffer with wrapping and stuffing, should check that
* we don't get tx buffer overflow.
*/
int async_wrap_skb(struct sk_buff *skb, __u8 *tx_buff, int buffsize)
{
struct irda_skb_cb *cb = (struct irda_skb_cb *) skb->cb;
int xbofs;
int i;
int n;
union {
__u16 value;
__u8 bytes[2];
} fcs;
/* Initialize variables */
fcs.value = INIT_FCS;
n = 0;
/*
* Send XBOF's for required min. turn time and for the negotiated
* additional XBOFS
*/
if (cb->magic != LAP_MAGIC) {
/*
* This will happen for all frames sent from user-space.
* Nothing to worry about, but we set the default number of
* BOF's
*/
IRDA_DEBUG(1, "%s(), wrong magic in skb!\n", __FUNCTION__);
xbofs = 10;
} else
xbofs = cb->xbofs + cb->xbofs_delay;
IRDA_DEBUG(4, "%s(), xbofs=%d\n", __FUNCTION__, xbofs);
/* Check that we never use more than 115 + 48 xbofs */
if (xbofs > 163) {
IRDA_DEBUG(0, "%s(), too many xbofs (%d)\n", __FUNCTION__,
xbofs);
xbofs = 163;
}
memset(tx_buff + n, XBOF, xbofs);
n += xbofs;
/* Start of packet character BOF */
tx_buff[n++] = BOF;
/* Insert frame and calc CRC */
for (i=0; i < skb->len; i++) {
/*
* Check for the possibility of tx buffer overflow. We use
* bufsize-5 since the maximum number of bytes that can be
* transmitted after this point is 5.
*/
if(n >= (buffsize-5)) {
IRDA_ERROR("%s(), tx buffer overflow (n=%d)\n",
__FUNCTION__, n);
return n;
}
n += stuff_byte(skb->data[i], tx_buff+n);
fcs.value = irda_fcs(fcs.value, skb->data[i]);
}
/* Insert CRC in little endian format (LSB first) */
fcs.value = ~fcs.value;
#ifdef __LITTLE_ENDIAN
n += stuff_byte(fcs.bytes[0], tx_buff+n);
n += stuff_byte(fcs.bytes[1], tx_buff+n);
#else /* ifdef __BIG_ENDIAN */
n += stuff_byte(fcs.bytes[1], tx_buff+n);
n += stuff_byte(fcs.bytes[0], tx_buff+n);
#endif
tx_buff[n++] = EOF;
return n;
}
EXPORT_SYMBOL(async_wrap_skb);
/************************* FRAME UNWRAPPING *************************/
/*
* Unwrap and unstuff SIR frames
*
* Complete rewrite by Jean II :
* More inline, faster, more compact, more logical. Jean II
* (16 bytes on P6 200MHz, old 5 to 7 us, new 4 to 6 us)
* (24 bytes on P6 200MHz, old 9 to 10 us, new 7 to 8 us)
* (for reference, 115200 b/s is 1 byte every 69 us)
* And reduce wrapper.o by ~900B in the process ;-)
*
* Then, we have the addition of ZeroCopy, which is optional
* (i.e. the driver must initiate it) and improve final processing.
* (2005 B frame + EOF on P6 200MHz, without 30 to 50 us, with 10 to 25 us)
*
* Note : at FIR and MIR, HDLC framing is used and usually handled
* by the controller, so we come here only for SIR... Jean II
*/
/*
* We can also choose where we want to do the CRC calculation. We can
* do it "inline", as we receive the bytes, or "postponed", when
* receiving the End-Of-Frame.
* (16 bytes on P6 200MHz, inlined 4 to 6 us, postponed 4 to 5 us)
* (24 bytes on P6 200MHz, inlined 7 to 8 us, postponed 5 to 7 us)
* With ZeroCopy :
* (2005 B frame on P6 200MHz, inlined 10 to 25 us, postponed 140 to 180 us)
* Without ZeroCopy :
* (2005 B frame on P6 200MHz, inlined 30 to 50 us, postponed 150 to 180 us)
* (Note : numbers taken with irq disabled)
*
* From those numbers, it's not clear which is the best strategy, because
* we end up running through a lot of data one way or another (i.e. cache
* misses). I personally prefer to avoid the huge latency spike of the
* "postponed" solution, because it come just at the time when we have
* lot's of protocol processing to do and it will hurt our ability to
* reach low link turnaround times... Jean II
*/
//#define POSTPONE_RX_CRC
/*
* Function async_bump (buf, len, stats)
*
* Got a frame, make a copy of it, and pass it up the stack! We can try
* to inline it since it's only called from state_inside_frame
*/
static inline void
async_bump(struct net_device *dev,
struct net_device_stats *stats,
iobuff_t *rx_buff)
{
struct sk_buff *newskb;
struct sk_buff *dataskb;
int docopy;
/* Check if we need to copy the data to a new skb or not.
* If the driver doesn't use ZeroCopy Rx, we have to do it.
* With ZeroCopy Rx, the rx_buff already point to a valid
* skb. But, if the frame is small, it is more efficient to
* copy it to save memory (copy will be fast anyway - that's
* called Rx-copy-break). Jean II */
docopy = ((rx_buff->skb == NULL) ||
(rx_buff->len < IRDA_RX_COPY_THRESHOLD));
/* Allocate a new skb */
newskb = dev_alloc_skb(docopy ? rx_buff->len + 1 : rx_buff->truesize);
if (!newskb) {
stats->rx_dropped++;
/* We could deliver the current skb if doing ZeroCopy Rx,
* but this would stall the Rx path. Better drop the
* packet... Jean II */
return;
}
/* Align IP header to 20 bytes (i.e. increase skb->data)
* Note this is only useful with IrLAN, as PPP has a variable
* header size (2 or 1 bytes) - Jean II */
skb_reserve(newskb, 1);
if(docopy) {
/* Copy data without CRC (lenght already checked) */
skb_copy_to_linear_data(newskb, rx_buff->data,
rx_buff->len - 2);
/* Deliver this skb */
dataskb = newskb;
} else {
/* We are using ZeroCopy. Deliver old skb */
dataskb = rx_buff->skb;
/* And hook the new skb to the rx_buff */
rx_buff->skb = newskb;
rx_buff->head = newskb->data; /* NOT newskb->head */
//printk(KERN_DEBUG "ZeroCopy : len = %d, dataskb = %p, newskb = %p\n", rx_buff->len, dataskb, newskb);
}
/* Set proper length on skb (without CRC) */
skb_put(dataskb, rx_buff->len - 2);
/* Feed it to IrLAP layer */
dataskb->dev = dev;
skb_reset_mac_header(dataskb);
dataskb->protocol = htons(ETH_P_IRDA);
netif_rx(dataskb);
stats->rx_packets++;
stats->rx_bytes += rx_buff->len;
/* Clean up rx_buff (redundant with async_unwrap_bof() ???) */
rx_buff->data = rx_buff->head;
rx_buff->len = 0;
}
/*
* Function async_unwrap_bof(dev, byte)
*
* Handle Beginning Of Frame character received within a frame
*
*/
static inline void
async_unwrap_bof(struct net_device *dev,
struct net_device_stats *stats,
iobuff_t *rx_buff, __u8 byte)
{
switch(rx_buff->state) {
case LINK_ESCAPE:
case INSIDE_FRAME:
/* Not supposed to happen, the previous frame is not
* finished - Jean II */
IRDA_DEBUG(1, "%s(), Discarding incomplete frame\n",
__FUNCTION__);
stats->rx_errors++;
stats->rx_missed_errors++;
irda_device_set_media_busy(dev, TRUE);
break;
case OUTSIDE_FRAME:
case BEGIN_FRAME:
default:
/* We may receive multiple BOF at the start of frame */
break;
}
/* Now receiving frame */
rx_buff->state = BEGIN_FRAME;
rx_buff->in_frame = TRUE;
/* Time to initialize receive buffer */
rx_buff->data = rx_buff->head;
rx_buff->len = 0;
rx_buff->fcs = INIT_FCS;
}
/*
* Function async_unwrap_eof(dev, byte)
*
* Handle End Of Frame character received within a frame
*
*/
static inline void
async_unwrap_eof(struct net_device *dev,
struct net_device_stats *stats,
iobuff_t *rx_buff, __u8 byte)
{
#ifdef POSTPONE_RX_CRC
int i;
#endif
switch(rx_buff->state) {
case OUTSIDE_FRAME:
/* Probably missed the BOF */
stats->rx_errors++;
stats->rx_missed_errors++;
irda_device_set_media_busy(dev, TRUE);
break;
case BEGIN_FRAME:
case LINK_ESCAPE:
case INSIDE_FRAME:
default:
/* Note : in the case of BEGIN_FRAME and LINK_ESCAPE,
* the fcs will most likely not match and generate an
* error, as expected - Jean II */
rx_buff->state = OUTSIDE_FRAME;
rx_buff->in_frame = FALSE;
#ifdef POSTPONE_RX_CRC
/* If we haven't done the CRC as we receive bytes, we
* must do it now... Jean II */
for(i = 0; i < rx_buff->len; i++)
rx_buff->fcs = irda_fcs(rx_buff->fcs,
rx_buff->data[i]);
#endif
/* Test FCS and signal success if the frame is good */
if (rx_buff->fcs == GOOD_FCS) {
/* Deliver frame */
async_bump(dev, stats, rx_buff);
break;
} else {
/* Wrong CRC, discard frame! */
irda_device_set_media_busy(dev, TRUE);
IRDA_DEBUG(1, "%s(), crc error\n", __FUNCTION__);
stats->rx_errors++;
stats->rx_crc_errors++;
}
break;
}
}
/*
* Function async_unwrap_ce(dev, byte)
*
* Handle Character Escape character received within a frame
*
*/
static inline void
async_unwrap_ce(struct net_device *dev,
struct net_device_stats *stats,
iobuff_t *rx_buff, __u8 byte)
{
switch(rx_buff->state) {
case OUTSIDE_FRAME:
/* Activate carrier sense */
irda_device_set_media_busy(dev, TRUE);
break;
case LINK_ESCAPE:
IRDA_WARNING("%s: state not defined\n", __FUNCTION__);
break;
case BEGIN_FRAME:
case INSIDE_FRAME:
default:
/* Stuffed byte coming */
rx_buff->state = LINK_ESCAPE;
break;
}
}
/*
* Function async_unwrap_other(dev, byte)
*
* Handle other characters received within a frame
*
*/
static inline void
async_unwrap_other(struct net_device *dev,
struct net_device_stats *stats,
iobuff_t *rx_buff, __u8 byte)
{
switch(rx_buff->state) {
/* This is on the critical path, case are ordered by
* probability (most frequent first) - Jean II */
case INSIDE_FRAME:
/* Must be the next byte of the frame */
if (rx_buff->len < rx_buff->truesize) {
rx_buff->data[rx_buff->len++] = byte;
#ifndef POSTPONE_RX_CRC
rx_buff->fcs = irda_fcs(rx_buff->fcs, byte);
#endif
} else {
IRDA_DEBUG(1, "%s(), Rx buffer overflow, aborting\n",
__FUNCTION__);
rx_buff->state = OUTSIDE_FRAME;
}
break;
case LINK_ESCAPE:
/*
* Stuffed char, complement bit 5 of byte
* following CE, IrLAP p.114
*/
byte ^= IRDA_TRANS;
if (rx_buff->len < rx_buff->truesize) {
rx_buff->data[rx_buff->len++] = byte;
#ifndef POSTPONE_RX_CRC
rx_buff->fcs = irda_fcs(rx_buff->fcs, byte);
#endif
rx_buff->state = INSIDE_FRAME;
} else {
IRDA_DEBUG(1, "%s(), Rx buffer overflow, aborting\n",
__FUNCTION__);
rx_buff->state = OUTSIDE_FRAME;
}
break;
case OUTSIDE_FRAME:
/* Activate carrier sense */
if(byte != XBOF)
irda_device_set_media_busy(dev, TRUE);
break;
case BEGIN_FRAME:
default:
rx_buff->data[rx_buff->len++] = byte;
#ifndef POSTPONE_RX_CRC
rx_buff->fcs = irda_fcs(rx_buff->fcs, byte);
#endif
rx_buff->state = INSIDE_FRAME;
break;
}
}
/*
* Function async_unwrap_char (dev, rx_buff, byte)
*
* Parse and de-stuff frame received from the IrDA-port
*
* This is the main entry point for SIR drivers.
*/
void async_unwrap_char(struct net_device *dev,
struct net_device_stats *stats,
iobuff_t *rx_buff, __u8 byte)
{
switch(byte) {
case CE:
async_unwrap_ce(dev, stats, rx_buff, byte);
break;
case BOF:
async_unwrap_bof(dev, stats, rx_buff, byte);
break;
case EOF:
async_unwrap_eof(dev, stats, rx_buff, byte);
break;
default:
async_unwrap_other(dev, stats, rx_buff, byte);
break;
}
}
EXPORT_SYMBOL(async_unwrap_char);
| {
"content_hash": "ba856d2029b57659e57937ccf925f04f",
"timestamp": "",
"source": "github",
"line_count": 466,
"max_line_length": 105,
"avg_line_length": 26.233905579399142,
"alnum_prop": 0.6386912065439673,
"repo_name": "ut-osa/laminar",
"id": "a7a7f191f1a86c94437b464f02fdaa5097787ba0",
"size": "13396",
"binary": false,
"copies": "43",
"ref": "refs/heads/master",
"path": "linux-2.6.22.6/net/irda/wrapper.c",
"mode": "33188",
"license": "bsd-3-clause",
"language": [
{
"name": "ASP",
"bytes": "4526"
},
{
"name": "Assembly",
"bytes": "7753785"
},
{
"name": "Awk",
"bytes": "5239"
},
{
"name": "Bison",
"bytes": "75151"
},
{
"name": "C",
"bytes": "209779557"
},
{
"name": "C++",
"bytes": "5954668"
},
{
"name": "CSS",
"bytes": "11885"
},
{
"name": "Java",
"bytes": "12132154"
},
{
"name": "Makefile",
"bytes": "731243"
},
{
"name": "Objective-C",
"bytes": "564040"
},
{
"name": "Perl",
"bytes": "196100"
},
{
"name": "Python",
"bytes": "11786"
},
{
"name": "Ruby",
"bytes": "3219"
},
{
"name": "Scala",
"bytes": "12158"
},
{
"name": "Scilab",
"bytes": "22980"
},
{
"name": "Shell",
"bytes": "205177"
},
{
"name": "TeX",
"bytes": "62636"
},
{
"name": "UnrealScript",
"bytes": "20822"
},
{
"name": "XSLT",
"bytes": "6544"
}
],
"symlink_target": ""
} |
// Copyright 2022 Google LLC
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package com.google.api.ads.admanager.jaxws.v202202;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
/**
*
* A {@code Creative} that contains Ad Manager hosted audio ads and is served via VAST XML. This
* creative is read-only.
*
*
* <p>Java class for AudioCreative complex type.
*
* <p>The following schema fragment specifies the expected content contained within this class.
*
* <pre>
* <complexType name="AudioCreative">
* <complexContent>
* <extension base="{https://www.google.com/apis/ads/publisher/v202202}BaseAudioCreative">
* <sequence>
* <element name="audioSourceUrl" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
* </pre>
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "AudioCreative", propOrder = {
"audioSourceUrl"
})
public class AudioCreative
extends BaseAudioCreative
{
protected String audioSourceUrl;
/**
* Gets the value of the audioSourceUrl property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAudioSourceUrl() {
return audioSourceUrl;
}
/**
* Sets the value of the audioSourceUrl property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAudioSourceUrl(String value) {
this.audioSourceUrl = value;
}
}
| {
"content_hash": "9c43e2de65a4e4675cb79a147fe4c7e7",
"timestamp": "",
"source": "github",
"line_count": 81,
"max_line_length": 108,
"avg_line_length": 27.604938271604937,
"alnum_prop": 0.6592128801431127,
"repo_name": "googleads/googleads-java-lib",
"id": "51eaa448da3676eaba834a51f3d98cafacb6c046",
"size": "2236",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "modules/dfp_appengine/src/main/java/com/google/api/ads/admanager/jaxws/v202202/AudioCreative.java",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "Java",
"bytes": "81068791"
}
],
"symlink_target": ""
} |
const assert = require('assert');
const Searcher = require('../resources/assets/js/Searcher.js');
const Board = require('../resources/assets/js/Board.js');
const Eventer = require('../resources/assets/js/Eventer.js');
const Championship = require('../resources/assets/js/Championship.js');
const ChampionshipMatch = require('../resources/assets/js/ChampionshipMatch.js');
const StrategyIterator = require('../resources/assets/js/StrategyIterator.js');
const InfiniteJest = require('./support/InfiniteJest.js');
const Loser = require('./support/Loser.js');
const ClassicWhos = require('../resources/assets/js/ClassicWhos.js');
const SayAnything = require('../resources/assets/js/strategies/SayAnything.js');
const _ = require('lodash');
describe('Searcher.And', function() {
describe('#constructor', function() {
it('should not error', function() {
new Searcher.And([]);
// no exception
});
});
describe('#test', function() {
it('should succeed on one', function() {
var and = new Searcher.And([/test/]);
assert.equal(true, and.test("test"));
});
it('should test false on one', function() {
var and = new Searcher.And([/test/]);
assert.equal(false, and.test("nope"));
});
it('should succeed on two', function() {
var and = new Searcher.And([/test/, /ster/]);
assert.equal(true, and.test("tester"));
});
it('should test false on first of two', function() {
var and = new Searcher.And([/test/, /ster/]);
assert.equal(false, and.test("napster"));
});
it('should test false on second of two', function() {
var and = new Searcher.And([/test/, /ster/]);
assert.equal(false, and.test("nu-test"));
});
it('should test false on two', function() {
var and = new Searcher.And([/test/, /ster/]);
assert.equal(false, and.test("nope"));
});
});
describe('Searcher.and', function() {
it('should succeed on two', function() {
var and = Searcher.and([/test/, /ster/]);
assert.equal(true, and.test("tester"));
});
});
});
describe('Searcher.Or', function() {
describe('#constructor', function() {
it('should not error', function() {
new Searcher.Or([]);
// no exception
});
});
describe('#test', function() {
it('should succeed on one', function() {
var or = new Searcher.Or([/test/]);
assert.equal(true, or.test("test"));
});
it('should test false on one', function() {
var or = new Searcher.Or([/test/]);
assert.equal(false, or.test("nope"));
});
it('should succeed on two', function() {
var or = new Searcher.Or([/test/, /ster/]);
assert.equal(true, or.test("tester"));
});
it('should test true on first of two', function() {
var or = new Searcher.Or([/test/, /ster/]);
assert.equal(true, or.test("napster"));
});
it('should test true on second of two', function() {
var or = new Searcher.Or([/test/, /ster/]);
assert.equal(true, or.test("nu-test"));
});
it('should test false on two', function() {
var or = new Searcher.Or([/test/, /ster/]);
assert.equal(false, or.test("nope"));
});
});
describe('Searcher.or', function() {
it('should succeed on two', function() {
var or = Searcher.or([/test/, /ster/]);
assert.equal(true, or.test("tester"));
});
});
});
describe('Searcher', function() {
describe('#where', function() {
it('should return itself', function() {
var searcher = new Searcher();
assert.equal(searcher, searcher.where('x', 1));
});
});
describe('#where (static)', function() {
it('should return itself', function() {
assert.equal(true, Searcher.where('x', 1) instanceof Searcher);
});
});
describe('#test()', function() {
it('should succeed on one', function() {
var searcher = Searcher.where('x', 1);
assert.equal(true, searcher.test({x: 1}));
});
it('should test false on one', function() {
var searcher = Searcher.where('x', 1);
assert.equal(false, searcher.test({x: 2}));
});
it('should succeed on two', function() {
var searcher = Searcher.where('x', 1).where('y', 'ABC');
assert.equal(true, searcher.test({x: 1, y: 'ABC'}));
});
it('should test false on first of two', function() {
var searcher = Searcher.where('x', 1).where('y', 'ABC');
assert.equal(false, searcher.test({x: 2, y: 'ABC'}));
});
it('should test false on second of two', function() {
var searcher = Searcher.where('x', 1).where('y', 'ABC');
assert.equal(false, searcher.test({x: 1, y: 'XYZ'}));
});
it('should test false on two', function() {
var searcher = Searcher.where('x', 1).where('y', 'ABC');
assert.equal(false, searcher.test({x: 2, y: 'XYZ'}));
});
});
describe('#push', function() {
it('should return this', function() {
var searcher = new Searcher();
assert.equal(searcher, searcher.push(/test/));
});
});
describe('#push (static)', function() {
it('should return this', function() {
assert.equal(true, Searcher.push(/test/) instanceof Searcher);
});
});
describe('#push && test', function() {
it('should run test on the object once', function() {
var tested = 0;
Searcher.push({test(){ tested++ }}).test({});
assert.equal(1, tested);
});
});
});
describe('Board', function() {
describe('#constructor', function() {
it('should instantiate', function() {
new Board();
});
it('should start with all remaining', function() {
var b = new Board(['x', 'y', 'z']);
assert.equal(b.remaining.length, 3);
assert.equal(b.rejected.length, 0);
});
});
describe('rejectMatches', function() {
it('should reject 0', function() {
var b = new Board(['x', 'y', 'z']);
b.rejectMatches(/a/);
assert.equal(b.remaining.length, 3);
assert.equal(b.rejected.length, 0);
});
it('should reject 1', function() {
var b = new Board(['x', 'y', 'z']);
b.rejectMatches(/z/);
assert.equal(b.remaining.length, 2);
assert.equal(b.rejected.length, 1);
});
it('should reject 2', function() {
var b = new Board(['x', 'y', 'z']);
b.rejectMatches(/[yz]/);
assert.equal(b.remaining.length, 1);
assert.equal(b.rejected.length, 2);
});
it('should reject all 3', function() {
var b = new Board(['x', 'y', 'z']);
b.rejectMatches(/./);
assert.equal(b.remaining.length, 0);
assert.equal(b.rejected.length, 3);
});
});
describe('rejectMisses', function() {
it('should reject all', function() {
var b = new Board(['x', 'y', 'z']);
b.rejectMisses(/a/);
assert.equal(b.remaining.length, 0);
assert.equal(b.rejected.length, 3);
});
it('should reject 2', function() {
var b = new Board(['x', 'y', 'z']);
b.rejectMisses(/z/);
assert.equal(b.remaining.length, 1);
assert.equal(b.rejected.length, 2);
});
it('should reject 1', function() {
var b = new Board(['x', 'y', 'z']);
b.rejectMisses(/[yz]/);
assert.equal(b.remaining.length, 2);
assert.equal(b.rejected.length, 1);
});
it('should reject 0', function() {
var b = new Board(['x', 'y', 'z']);
b.rejectMisses(/./);
assert.equal(b.remaining.length, 3);
assert.equal(b.rejected.length, 0);
});
});
});
describe('Eventer', function() {
describe('#construct', function() {
it('should instantiate', function() {
new Eventer();
});
});
describe('#on', function() {
it('should accept a closure', function() {
new Eventer().on(function(){});
});
it('should return self', function() {
var ev = new Eventer();
assert.equal(ev.on(function(){}), ev);
});
});
describe('#fire', function() {
it('should do nothing', function() {
new Eventer().fire();
});
it('should fire one closure once', function() {
var ran = 0;
var ev = new Eventer().on(function(){ ran++ });
ev.fire();
assert.equal(ran, 1);
});
it('should fire one closure twice', function() {
var ran = 0;
var ev = new Eventer().on(function(){ ran++ });
ev.fire();
ev.fire();
assert.equal(ran, 2);
});
it('should fire two closures once each', function() {
var ran = 0;
var ev = new Eventer();
ev.on(function(){ ran++ });
ev.on(function(){ ran++ });
ev.fire();
assert.equal(ran, 2);
});
it('should pass args', function() {
var ran = '';
var ev = new Eventer().on(function(a, b){ ran = a + " " + b });
ev.fire('hello', 'world');
assert.equal(ran, 'hello world');
});
});
});
describe('ChampionshipMatch', function() {
describe('#instantiate', function() {
it('should instantiate', function() {
var m = new ChampionshipMatch({}, {}, []);
assert.equal(m.finished, false);
});
});
describe('#run & stop', function() {
it('should runStep a few times and then stop', function(done) {
var m = new ChampionshipMatch({}, {}, []);
var ran = 0;
m.runStep = function() { ran++ };
m.run();
setTimeout(function() {
m.stop();
assert(ran > 0);
assert.equal(m.runner, null);
done();
}, 10);
});
it('should runStep a few times, pause, runStep a few more times, then stop', function(done) {
var m = new ChampionshipMatch({}, {}, []);
var ran = 0;
var firstRun = 0;
m.runStep = function() { ran++ };
m.run();
setTimeout(function() {
m.stop();
assert(ran > 0);
firstRun = ran;
assert.equal(m.runner, null);
m.run();
}, 10);
setTimeout(function() {
m.stop();
assert(ran > firstRun);
assert.equal(m.runner, null);
done();
}, 20);
});
it('should runStep until it quits for too many turns', function(done) {
var m = new ChampionshipMatch(InfiniteJest, InfiniteJest, [], 10);
m.run().onError(function() {
assert.equal(m.turns, 11);
done();
});
});
});
describe('#runStep', function() {
it('should get a move and handle it', function() {
var caughtMove;
var m = new ChampionshipMatch({}, {}, []);
m.otherInfo = function(){};
m.buildStrategy = function() {
return {
move() {
return 'XXXX';
}
};
};
m.handleMove = function(move) {
caughtMove = move;
};
m.runStep();
assert.equal(caughtMove, 'XXXX');
});
it('should end match on exceptions', function() {
var caughtMove;
var m = new ChampionshipMatch({}, {}, ClassicWhos);
m.otherInfo = function(){};
m.buildStrategy = function() {
return {
move() {
throw new Error();
}
};
};
m.runStep();
assert(m.finished);
});
});
describe('#buildStrategy', function() {
it('should new up a class', function() {
class X{}
var m = new ChampionshipMatch({}, {}, []);
var x = m.buildStrategy(X);
assert(x instanceof X);
});
});
describe('#switch', function() {
it('should switch out the current player with the other one', function() {
var a = {};
var b = {};
var m = new ChampionshipMatch(a, b, []);
assert.equal(m.current.player, a);
m.switch();
assert.equal(m.current.player, b);
m.switch();
assert.equal(m.current.player, a);
});
});
describe('#handleMove', function() {
it('should call finish()', function() {
var m = new ChampionshipMatch({}, {}, []);
var finished = false;
m.finish = function() { finished = true };
m.handleMove("string");
assert(finished);
});
it('should reject misses', function() {
var m = new ChampionshipMatch({}, {}, []);
var ran = 0;
m.a.board.rejectMisses = function() { ran++ };
m.handleMove({
test() {
return true;
}
});
assert(ran, 1);
});
it('should reject matches', function() {
var m = new ChampionshipMatch({}, {}, []);
var ran = 0;
m.a.board.rejectMatches = function() { ran++ };
m.handleMove({
test() {
return false;
}
});
assert(ran, 1);
});
it('should do nothing with non-data', function() {
var m = new ChampionshipMatch({}, {}, ClassicWhos);
m.handleMove(null);
assert(m.a.board.remaining.length, ClassicWhos.length);
assert(m.b.board.remaining.length, ClassicWhos.length);
});
});
describe('#finish', function() {
it('should finish with player A the winner', function() {
var m = new ChampionshipMatch({}, {}, ClassicWhos);
m.b.who = {name: 'ABC'};
var result;
m.onDone(function(doneData) {
result = doneData;
});
m.finish('ABC');
assert(m.finished);
assert.equal(result.turns, 0);
assert.equal(result.winner, 0);
assert(result.players instanceof Array);
});
it('should finish with player B the winner', function() {
var m = new ChampionshipMatch({}, {}, ClassicWhos);
m.b.who = 'XYZ';
var result;
m.onDone(function(doneData) {
result = doneData;
});
m.finish('ABC');
assert(m.finished);
assert.equal(result.turns, 0);
assert.equal(result.winner, 1);
assert(result.players instanceof Array);
});
});
describe('#otherInfo', function() {
it('should give the info of player b', function() {
var b = {};
var m = new ChampionshipMatch({}, b, ['x', 'y', 'z']);
m.b.board.remaining = ['x', 'y'];
m.b.board.rejected = ['z'];
var info = m.otherInfo();
assert(info instanceof Object);
assert.equal(info.player, b);
assert.equal(info.board.remaining.length, 2);
assert.equal(info.board.rejected.length, 1);
});
});
describe('#currentInfo', function() {
it('shold give the info of player a', function() {
var a = {};
var m = new ChampionshipMatch(a, {}, ['x', 'y', 'z']);
m.a.board.remaining = ['x', 'y'];
m.a.board.rejected = ['z'];
m.a.who = 'z';
var info = m.currentInfo();
assert(info instanceof Object);
assert.equal(info.player, a);
assert.equal(info.board.remaining.length, 2);
assert.equal(info.board.rejected.length, 1);
assert.equal(info.who, 'z');
});
});
describe('#getReport', function() {
it('should say that a is winning', function() {
var m = new ChampionshipMatch({}, {}, ['x', 'y', 'z']);
m.a.board.rejected = ['x'];
m.a.board.remaining = ['y', 'z'];
var report = m.getReport();
assert(report instanceof Object);
assert.equal(report.winning, 0);
assert.equal(report.progress, 1/6);
assert(report.players instanceof Array);
});
it('should say that b is winning', function() {
var m = new ChampionshipMatch({}, {}, ['x', 'y', 'z']);
m.b.board.rejected = ['x'];
m.b.board.remaining = ['y', 'z'];
var report = m.getReport();
assert(report instanceof Object);
assert.equal(report.winning, 1);
assert.equal(report.progress, 1/6);
assert(report.players instanceof Array);
});
it('should say that b is winning because of tie and b has next turn', function() {
var m = new ChampionshipMatch({}, {}, ['x', 'y', 'z']);
var report = m.getReport();
assert(report instanceof Object);
assert.equal(report.winning, 1);
assert.equal(report.progress, 0);
assert(report.players instanceof Array);
});
});
});
describe('StrategyIterator', function() {
describe('#instantiate', function() {
it('builds', function() {
new StrategyIterator(['a', 'b']);
});
});
describe('#next', function() {
it('should give a,a; a,b; b,a; b,b; <null>', function() {
var it = new StrategyIterator(['a', 'b']);
var x, y;
[x, y] = it.next();
assert.equal('a', x);
assert.equal('a', y);
[x, y] = it.next();
assert.equal('a', x);
assert.equal('b', y);
[x, y] = it.next();
assert.equal('b', x);
assert.equal('a', y);
[x, y] = it.next();
assert.equal('b', x);
assert.equal('b', y);
assert(it.next() === null);
});
});
});
describe('Championship', function() {
describe('#instantiate', function() {
it('should instantiate', function() {
new Championship([]);
});
});
describe('#finish', function() {
it('should set finished and fire event', function() {
var c = new Championship([]);
var ran = 0;
c.onDone(function(){ ran++ });
c.finish();
assert(c.finished);
assert.equal(ran, 1);
});
});
describe('#nextCompetitors', function() {
it('should get one pair of competitors', function() {
var c = new Championship(['a']);
var competitors = c.nextCompetitors();
assert.equal(competitors[0], 'a');
assert.equal(competitors[1], 'a');
});
it('should get one pair of competitors, then none', function() {
var c = new Championship(['a']);
var competitors = c.nextCompetitors();
assert.equal(competitors[0], 'a');
assert.equal(competitors[1], 'a');
competitors = c.nextCompetitors();
assert(competitors === null);
});
it('should get one pair of competitors twice, then none', function() {
var c = new Championship(['a'], [], 2);
var competitors = c.nextCompetitors();
assert.equal(competitors[0], 'a');
assert.equal(competitors[1], 'a');
competitors = c.nextCompetitors();
assert.equal(competitors[0], 'a');
assert.equal(competitors[1], 'a');
competitors = c.nextCompetitors();
assert(competitors === null);
});
});
describe('run and stop, with InfiniteJest', function() {
it('should run for a while, then stop', function(done) {
var c = new Championship(
[InfiniteJest],
ClassicWhos
);
c.run();
setTimeout(() => {
c.stop();
done();
}, 10);
});
it('should run for a while, receive progress, then stop', function(done) {
var c = new Championship(
[InfiniteJest],
ClassicWhos
);
var progresses = [];
c.onProgress(function(progress){ progresses.push(progress) });
c.run();
setTimeout(() => {
c.stop();
assert(progresses.length > 0);
done();
}, 10);
});
});
describe('run and stop, with Loser', function() {
it('should run a quick match, then finish', function(done) {
var c = new Championship(
[Loser],
ClassicWhos
);
c.onDone(function(report){
assert(report instanceof Object);
assert.equal(report.rounds, 1);
assert(report.results instanceof Array);
assert(report.results[0] instanceof Object);
assert.equal(report.results[0].turns, 1);
assert.equal(report.results[0].winner, 1);
assert.equal(report.results[0].reason, ChampionshipMatch.REASON_INCORRECT_ANSWER);
done();
});
c.run();
});
it('should run 100 matches, then finish', function(done) {
var c = new Championship(
[Loser],
ClassicWhos,
100
);
c.onDone(function(report){
assert(report instanceof Object);
assert.equal(report.rounds, 100);
assert(report.results instanceof Array);
assert.equal(report.results.length, 100);
report.results.map(function(result) {
assert(result instanceof Object);
assert.equal(result.turns, 1);
assert.equal(result.winner, 1);
assert.equal(result.reason, ChampionshipMatch.REASON_INCORRECT_ANSWER);
});
done();
});
c.run();
});
});
});
describe('SayAnything', function() {
describe('Winner every time', function() {
it('should pick the only solution that does not have the same name', function() {
var whos = ClassicWhos.slice(0, 2);
var answer = new SayAnything().move({who: whos[0], board: {remaining: whos}}, {}, function(){});
assert.equal(answer, whos[1].name);
});
});
describe('Winner half the time', function() {
it('should pick one of two solutions that do not have the same name', function() {
var whos = ClassicWhos.slice(0, 3);
var correct = 0;
for (var i = 0; i < 100; i++) {
var answer = new SayAnything().move({who: whos[0], board: {remaining: whos}}, {}, function(){});
if (answer == whos[1].name) {
correct++;
}
}
assert(correct > 0);
assert(correct < 100);
});
});
});
describe('ClassicWhos', function() {
describe('Check fields', function() {
it('should have only expected fields', function() {
var whoKeys = ClassicWhos.map((who) => Object.keys(who));
var keys = _(whoKeys).flatten().unique().value();
var approved = [
'bald',
'bangs',
'beard',
'earring_color',
'earrings',
'eye_color',
'gender',
'glasses',
'hair_color',
'hat',
'hat_color',
'mustache',
'name',
];
var newProps = _.difference(keys, approved);
assert.equal(newProps.length, 0, 'There are new properties in the ClassicWhos array: ' + newProps.join(', ') + '. Maybe you misspelled it, or maybe you just need to add it to the approved list in the test.js');
});
it('should have expected proportions of fields', function() {
var expectedProportions = {
bald: 5,
bangs: 4,
beard: 4,
earring_color: 3,
earrings: 3,
eye_color: 24,
gender: 24,
glasses: 5,
hair_color: 24,
hat: 5,
hat_color: 5,
mustache: 5,
name: 24,
};
var proportions = {
bald: 0,
bangs: 0,
beard: 0,
earring_color: 0,
earrings: 0,
eye_color: 0,
gender: 0,
glasses: 0,
hair_color: 0,
hat: 0,
hat_color: 0,
mustache: 0,
name: 0,
};
for (var field in expectedProportions) {
ClassicWhos.map(who => {
if (who[field]) {
proportions[field]++;
}
});
assert.equal(proportions[field], expectedProportions[field], 'Unexpected number of individuals with ' + field + ' set. Check your Who list or check the test.js file.');
}
});
it('should have a certain number of ladies', function() {
const ladies = ClassicWhos.filter(who => Searcher.where('gender', 'female').test(who));
assert.equal(ladies.length, 5, 'Expected exactly 5 ladies, update either ClassicWhos or tests.js');
});
it('should have a certain number of gentlemen', function() {
const ladies = ClassicWhos.filter(who => Searcher.where('gender', 'male').test(who));
assert.equal(ladies.length, 19, 'Expected exactly 19 gentlemen, update either ClassicWhos or tests.js');
});
it('should have a certain number of each hair color', function() {
const hairColors = {
brown: 0,
white: 0,
blonde: 0,
red: 0,
black: 0,
};
ClassicWhos.map(who => hairColors[who.hair_color]++);
assert.equal(hairColors.brown, 4, 'Expected 4 brown haired people, check the ClassicWhos and the test.js file');
assert.equal(hairColors.white, 5, 'Expected 5 white haired people, check the ClassicWhos and the test.js file');
assert.equal(hairColors.blonde, 5, 'Expected 5 blonde haired people, check the ClassicWhos and the test.js file');
assert.equal(hairColors.red , 5, 'Expected 5 red haired people, check the ClassicWhos and the test.js file');
assert.equal(hairColors.black , 5, 'Expected 5 black haired people, check the ClassicWhos and the test.js file');
});
it('should have a certain number of blue-eyed folks', function() {
const ladies = ClassicWhos.filter(who => Searcher.where('eye_color', 'blue').test(who));
assert.equal(ladies.length, 5, 'Expected exactly 5 blue-eyed people, update either ClassicWhos or tests.js');
});
it('should have a certain number of brown-eyed folks', function() {
const ladies = ClassicWhos.filter(who => Searcher.where('eye_color', 'brown').test(who));
assert.equal(ladies.length, 19, 'Expected exactly 19 brown-eyed people, update either ClassicWhos or tests.js');
});
});
});
| {
"content_hash": "82975c35c34c4644fb0e70a775cab427",
"timestamp": "",
"source": "github",
"line_count": 771,
"max_line_length": 222,
"avg_line_length": 37.30609597924773,
"alnum_prop": 0.4824948718840177,
"repo_name": "dankuck/who-guessers",
"id": "b99d3cdb88e56b685bda40e86a37ffe945c2e553",
"size": "28763",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "test/test.js",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "HTML",
"bytes": "1450"
},
{
"name": "JavaScript",
"bytes": "64604"
},
{
"name": "Vue",
"bytes": "21728"
}
],
"symlink_target": ""
} |
namespace chromeos {
namespace {
#define ENUM_CASE(x) case x: return std::string(#x)
std::string IPConfigTypeAsString(IPConfigType type) {
switch (type) {
ENUM_CASE(IPCONFIG_TYPE_UNKNOWN);
ENUM_CASE(IPCONFIG_TYPE_IPV4);
ENUM_CASE(IPCONFIG_TYPE_IPV6);
ENUM_CASE(IPCONFIG_TYPE_DHCP);
ENUM_CASE(IPCONFIG_TYPE_BOOTP);
ENUM_CASE(IPCONFIG_TYPE_ZEROCONF);
ENUM_CASE(IPCONFIG_TYPE_DHCP6);
ENUM_CASE(IPCONFIG_TYPE_PPP);
}
NOTREACHED() << "Unhandled enum value " << type;
return std::string();
}
#undef ENUM_CASE
} // namespace
NetworkIPConfig::NetworkIPConfig(
const std::string& device_path, IPConfigType type,
const std::string& address, const std::string& netmask,
const std::string& gateway, const std::string& name_servers)
: device_path(device_path),
type(type),
address(address),
netmask(netmask),
gateway(gateway),
name_servers(name_servers) {
}
NetworkIPConfig::~NetworkIPConfig() {}
std::string NetworkIPConfig::ToString() const {
return std::string("path: ") + device_path
+ " type: " + IPConfigTypeAsString(type)
+ " address: " + address
+ " netmask: " + netmask
+ " gateway: " + gateway
+ " name_servers: " + name_servers;
}
} // namespace chromeos
| {
"content_hash": "d02e5b0ed564df7e7589e09833dac924",
"timestamp": "",
"source": "github",
"line_count": 45,
"max_line_length": 64,
"avg_line_length": 28.4,
"alnum_prop": 0.6580594679186228,
"repo_name": "leiferikb/bitpop-private",
"id": "4dd105e0f369a319a5fe08946193c6458099e930",
"size": "1536",
"binary": false,
"copies": "6",
"ref": "refs/heads/master",
"path": "chrome/browser/chromeos/cros/network_ip_config.cc",
"mode": "33188",
"license": "bsd-3-clause",
"language": [
{
"name": "AppleScript",
"bytes": "6973"
},
{
"name": "Arduino",
"bytes": "464"
},
{
"name": "Assembly",
"bytes": "1871"
},
{
"name": "C",
"bytes": "1800028"
},
{
"name": "C++",
"bytes": "76499582"
},
{
"name": "CSS",
"bytes": "803682"
},
{
"name": "Java",
"bytes": "1234788"
},
{
"name": "JavaScript",
"bytes": "21793252"
},
{
"name": "Objective-C",
"bytes": "5358744"
},
{
"name": "PHP",
"bytes": "97817"
},
{
"name": "Perl",
"bytes": "64410"
},
{
"name": "Python",
"bytes": "3017857"
},
{
"name": "Ruby",
"bytes": "650"
},
{
"name": "Shell",
"bytes": "322362"
},
{
"name": "XSLT",
"bytes": "418"
},
{
"name": "nesC",
"bytes": "12138"
}
],
"symlink_target": ""
} |
package njson
// JSON value parser state machine.
// Just about at the limit of what is reasonable to write by hand.
// Some parts are a bit tedious, but overall it nicely factors out the
// otherwise common code from the multiple scanning functions
// in this package (Compact, Indent, checkValid, nextValue, etc).
//
// This file starts with two simple examples using the scanner
// before diving into the scanner itself.
import "strconv"
// checkValid verifies that data is valid JSON-encoded data.
// scan is passed in for use by checkValid to avoid an allocation.
func checkValid(data []byte, scan *scanner) error {
scan.reset()
for _, c := range data {
scan.bytes++
if scan.step(scan, int(c)) == scanError {
return scan.err
}
}
if scan.eof() == scanError {
return scan.err
}
return nil
}
// nextValue splits data after the next whole JSON value,
// returning that value and the bytes that follow it as separate slices.
// scan is passed in for use by nextValue to avoid an allocation.
func nextValue(data []byte, scan *scanner) (value, rest []byte, err error) {
scan.reset()
for i, c := range data {
v := scan.step(scan, int(c))
if v >= scanEnd {
switch v {
case scanError:
return nil, nil, scan.err
case scanEnd:
return data[0:i], data[i:], nil
}
}
}
if scan.eof() == scanError {
return nil, nil, scan.err
}
return data, nil, nil
}
// A SyntaxError is a description of a JSON syntax error.
type SyntaxError struct {
msg string // description of error
Offset int64 // error occurred after reading Offset bytes
}
func (e *SyntaxError) Error() string { return e.msg }
// A scanner is a JSON scanning state machine.
// Callers call scan.reset() and then pass bytes in one at a time
// by calling scan.step(&scan, c) for each byte.
// The return value, referred to as an opcode, tells the
// caller about significant parsing events like beginning
// and ending literals, objects, and arrays, so that the
// caller can follow along if it wishes.
// The return value scanEnd indicates that a single top-level
// JSON value has been completed, *before* the byte that
// just got passed in. (The indication must be delayed in order
// to recognize the end of numbers: is 123 a whole value or
// the beginning of 12345e+6?).
type scanner struct {
// The step is a func to be called to execute the next transition.
// Also tried using an integer constant and a single func
// with a switch, but using the func directly was 10% faster
// on a 64-bit Mac Mini, and it's nicer to read.
step func(*scanner, int) int
// Reached end of top-level value.
endTop bool
// Stack of what we're in the middle of - array values, object keys, object values.
parseState []int
// Error that happened, if any.
err error
// 1-byte redo (see undo method)
redo bool
redoCode int
redoState func(*scanner, int) int
// total bytes consumed, updated by decoder.Decode
bytes int64
}
// These values are returned by the state transition functions
// assigned to scanner.state and the method scanner.eof.
// They give details about the current state of the scan that
// callers might be interested to know about.
// It is okay to ignore the return value of any particular
// call to scanner.state: if one call returns scanError,
// every subsequent call will return scanError too.
const (
// Continue.
scanContinue = iota // uninteresting byte
scanBeginLiteral // end implied by next result != scanContinue
scanBeginObject // begin object
scanObjectKey // just finished object key (string)
scanObjectValue // just finished non-last object value
scanEndObject // end object (implies scanObjectValue if possible)
scanBeginArray // begin array
scanArrayValue // just finished array value
scanEndArray // end array (implies scanArrayValue if possible)
scanSkipSpace // space byte; can skip; known to be last "continue" result
// Stop.
scanEnd // top-level value ended *before* this byte; known to be first "stop" result
scanError // hit an error, scanner.err.
)
// These values are stored in the parseState stack.
// They give the current state of a composite value
// being scanned. If the parser is inside a nested value
// the parseState describes the nested state, outermost at entry 0.
const (
parseObjectKey = iota // parsing object key (before colon)
parseObjectValue // parsing object value (after colon)
parseArrayValue // parsing array value
)
// reset prepares the scanner for use.
// It must be called before calling s.step.
func (s *scanner) reset() {
s.step = stateBeginValue
s.parseState = s.parseState[0:0]
s.err = nil
s.redo = false
s.endTop = false
}
// eof tells the scanner that the end of input has been reached.
// It returns a scan status just as s.step does.
func (s *scanner) eof() int {
if s.err != nil {
return scanError
}
if s.endTop {
return scanEnd
}
s.step(s, ' ')
if s.endTop {
return scanEnd
}
if s.err == nil {
s.err = &SyntaxError{"unexpected end of JSON input", s.bytes}
}
return scanError
}
// pushParseState pushes a new parse state p onto the parse stack.
func (s *scanner) pushParseState(p int) {
s.parseState = append(s.parseState, p)
}
// popParseState pops a parse state (already obtained) off the stack
// and updates s.step accordingly.
func (s *scanner) popParseState() {
n := len(s.parseState) - 1
s.parseState = s.parseState[0:n]
s.redo = false
if n == 0 {
s.step = stateEndTop
s.endTop = true
} else {
s.step = stateEndValue
}
}
func isSpace(c rune) bool {
return c == ' ' || c == '\t' || c == '\r' || c == '\n'
}
// stateBeginValueOrEmpty is the state after reading `[`.
func stateBeginValueOrEmpty(s *scanner, c int) int {
if c <= ' ' && isSpace(rune(c)) {
return scanSkipSpace
}
if c == ']' {
return stateEndValue(s, c)
}
return stateBeginValue(s, c)
}
// stateBeginValue is the state at the beginning of the input.
func stateBeginValue(s *scanner, c int) int {
if c <= ' ' && isSpace(rune(c)) {
return scanSkipSpace
}
switch c {
case '{':
s.step = stateBeginStringOrEmpty
s.pushParseState(parseObjectKey)
return scanBeginObject
case '[':
s.step = stateBeginValueOrEmpty
s.pushParseState(parseArrayValue)
return scanBeginArray
case '"':
s.step = stateInString
return scanBeginLiteral
case '-':
s.step = stateNeg
return scanBeginLiteral
case '0': // beginning of 0.123
s.step = state0
return scanBeginLiteral
case 't': // beginning of true
s.step = stateT
return scanBeginLiteral
case 'f': // beginning of false
s.step = stateF
return scanBeginLiteral
case 'n': // beginning of null
s.step = stateN
return scanBeginLiteral
}
if '1' <= c && c <= '9' { // beginning of 1234.5
s.step = state1
return scanBeginLiteral
}
return s.error(c, "looking for beginning of value")
}
// stateBeginStringOrEmpty is the state after reading `{`.
func stateBeginStringOrEmpty(s *scanner, c int) int {
if c <= ' ' && isSpace(rune(c)) {
return scanSkipSpace
}
if c == '}' {
n := len(s.parseState)
s.parseState[n-1] = parseObjectValue
return stateEndValue(s, c)
}
return stateBeginString(s, c)
}
// stateBeginString is the state after reading `{"key": value,`.
func stateBeginString(s *scanner, c int) int {
if c <= ' ' && isSpace(rune(c)) {
return scanSkipSpace
}
if c == '"' {
s.step = stateInString
return scanBeginLiteral
}
return s.error(c, "looking for beginning of object key string")
}
// stateEndValue is the state after completing a value,
// such as after reading `{}` or `true` or `["x"`.
func stateEndValue(s *scanner, c int) int {
n := len(s.parseState)
if n == 0 {
// Completed top-level before the current byte.
s.step = stateEndTop
s.endTop = true
return stateEndTop(s, c)
}
if c <= ' ' && isSpace(rune(c)) {
s.step = stateEndValue
return scanSkipSpace
}
ps := s.parseState[n-1]
switch ps {
case parseObjectKey:
if c == ':' {
s.parseState[n-1] = parseObjectValue
s.step = stateBeginValue
return scanObjectKey
}
return s.error(c, "after object key")
case parseObjectValue:
if c == ',' {
s.parseState[n-1] = parseObjectKey
s.step = stateBeginString
return scanObjectValue
}
if c == '}' {
s.popParseState()
return scanEndObject
}
return s.error(c, "after object key:value pair")
case parseArrayValue:
if c == ',' {
s.step = stateBeginValue
return scanArrayValue
}
if c == ']' {
s.popParseState()
return scanEndArray
}
return s.error(c, "after array element")
}
return s.error(c, "")
}
// stateEndTop is the state after finishing the top-level value,
// such as after reading `{}` or `[1,2,3]`.
// Only space characters should be seen now.
func stateEndTop(s *scanner, c int) int {
if c != ' ' && c != '\t' && c != '\r' && c != '\n' {
// Complain about non-space byte on next call.
s.error(c, "after top-level value")
}
return scanEnd
}
// stateInString is the state after reading `"`.
func stateInString(s *scanner, c int) int {
if c == '"' {
s.step = stateEndValue
return scanContinue
}
if c == '\\' {
s.step = stateInStringEsc
return scanContinue
}
if c < 0x20 {
return s.error(c, "in string literal")
}
return scanContinue
}
// stateInStringEsc is the state after reading `"\` during a quoted string.
func stateInStringEsc(s *scanner, c int) int {
switch c {
case 'b', 'f', 'n', 'r', 't', '\\', '/', '"':
s.step = stateInString
return scanContinue
}
if c == 'u' {
s.step = stateInStringEscU
return scanContinue
}
return s.error(c, "in string escape code")
}
// stateInStringEscU is the state after reading `"\u` during a quoted string.
func stateInStringEscU(s *scanner, c int) int {
if '0' <= c && c <= '9' || 'a' <= c && c <= 'f' || 'A' <= c && c <= 'F' {
s.step = stateInStringEscU1
return scanContinue
}
// numbers
return s.error(c, "in \\u hexadecimal character escape")
}
// stateInStringEscU1 is the state after reading `"\u1` during a quoted string.
func stateInStringEscU1(s *scanner, c int) int {
if '0' <= c && c <= '9' || 'a' <= c && c <= 'f' || 'A' <= c && c <= 'F' {
s.step = stateInStringEscU12
return scanContinue
}
// numbers
return s.error(c, "in \\u hexadecimal character escape")
}
// stateInStringEscU12 is the state after reading `"\u12` during a quoted string.
func stateInStringEscU12(s *scanner, c int) int {
if '0' <= c && c <= '9' || 'a' <= c && c <= 'f' || 'A' <= c && c <= 'F' {
s.step = stateInStringEscU123
return scanContinue
}
// numbers
return s.error(c, "in \\u hexadecimal character escape")
}
// stateInStringEscU123 is the state after reading `"\u123` during a quoted string.
func stateInStringEscU123(s *scanner, c int) int {
if '0' <= c && c <= '9' || 'a' <= c && c <= 'f' || 'A' <= c && c <= 'F' {
s.step = stateInString
return scanContinue
}
// numbers
return s.error(c, "in \\u hexadecimal character escape")
}
// stateNeg is the state after reading `-` during a number.
func stateNeg(s *scanner, c int) int {
if c == '0' {
s.step = state0
return scanContinue
}
if '1' <= c && c <= '9' {
s.step = state1
return scanContinue
}
return s.error(c, "in numeric literal")
}
// state1 is the state after reading a non-zero integer during a number,
// such as after reading `1` or `100` but not `0`.
func state1(s *scanner, c int) int {
if '0' <= c && c <= '9' {
s.step = state1
return scanContinue
}
return state0(s, c)
}
// state0 is the state after reading `0` during a number.
func state0(s *scanner, c int) int {
if c == '.' {
s.step = stateDot
return scanContinue
}
if c == 'e' || c == 'E' {
s.step = stateE
return scanContinue
}
return stateEndValue(s, c)
}
// stateDot is the state after reading the integer and decimal point in a number,
// such as after reading `1.`.
func stateDot(s *scanner, c int) int {
if '0' <= c && c <= '9' {
s.step = stateDot0
return scanContinue
}
return s.error(c, "after decimal point in numeric literal")
}
// stateDot0 is the state after reading the integer, decimal point, and subsequent
// digits of a number, such as after reading `3.14`.
func stateDot0(s *scanner, c int) int {
if '0' <= c && c <= '9' {
s.step = stateDot0
return scanContinue
}
if c == 'e' || c == 'E' {
s.step = stateE
return scanContinue
}
return stateEndValue(s, c)
}
// stateE is the state after reading the mantissa and e in a number,
// such as after reading `314e` or `0.314e`.
func stateE(s *scanner, c int) int {
if c == '+' {
s.step = stateESign
return scanContinue
}
if c == '-' {
s.step = stateESign
return scanContinue
}
return stateESign(s, c)
}
// stateESign is the state after reading the mantissa, e, and sign in a number,
// such as after reading `314e-` or `0.314e+`.
func stateESign(s *scanner, c int) int {
if '0' <= c && c <= '9' {
s.step = stateE0
return scanContinue
}
return s.error(c, "in exponent of numeric literal")
}
// stateE0 is the state after reading the mantissa, e, optional sign,
// and at least one digit of the exponent in a number,
// such as after reading `314e-2` or `0.314e+1` or `3.14e0`.
func stateE0(s *scanner, c int) int {
if '0' <= c && c <= '9' {
s.step = stateE0
return scanContinue
}
return stateEndValue(s, c)
}
// stateT is the state after reading `t`.
func stateT(s *scanner, c int) int {
if c == 'r' {
s.step = stateTr
return scanContinue
}
return s.error(c, "in literal true (expecting 'r')")
}
// stateTr is the state after reading `tr`.
func stateTr(s *scanner, c int) int {
if c == 'u' {
s.step = stateTru
return scanContinue
}
return s.error(c, "in literal true (expecting 'u')")
}
// stateTru is the state after reading `tru`.
func stateTru(s *scanner, c int) int {
if c == 'e' {
s.step = stateEndValue
return scanContinue
}
return s.error(c, "in literal true (expecting 'e')")
}
// stateF is the state after reading `f`.
func stateF(s *scanner, c int) int {
if c == 'a' {
s.step = stateFa
return scanContinue
}
return s.error(c, "in literal false (expecting 'a')")
}
// stateFa is the state after reading `fa`.
func stateFa(s *scanner, c int) int {
if c == 'l' {
s.step = stateFal
return scanContinue
}
return s.error(c, "in literal false (expecting 'l')")
}
// stateFal is the state after reading `fal`.
func stateFal(s *scanner, c int) int {
if c == 's' {
s.step = stateFals
return scanContinue
}
return s.error(c, "in literal false (expecting 's')")
}
// stateFals is the state after reading `fals`.
func stateFals(s *scanner, c int) int {
if c == 'e' {
s.step = stateEndValue
return scanContinue
}
return s.error(c, "in literal false (expecting 'e')")
}
// stateN is the state after reading `n`.
func stateN(s *scanner, c int) int {
if c == 'u' {
s.step = stateNu
return scanContinue
}
return s.error(c, "in literal null (expecting 'u')")
}
// stateNu is the state after reading `nu`.
func stateNu(s *scanner, c int) int {
if c == 'l' {
s.step = stateNul
return scanContinue
}
return s.error(c, "in literal null (expecting 'l')")
}
// stateNul is the state after reading `nul`.
func stateNul(s *scanner, c int) int {
if c == 'l' {
s.step = stateEndValue
return scanContinue
}
return s.error(c, "in literal null (expecting 'l')")
}
// stateError is the state after reaching a syntax error,
// such as after reading `[1}` or `5.1.2`.
func stateError(s *scanner, c int) int {
return scanError
}
// error records an error and switches to the error state.
func (s *scanner) error(c int, context string) int {
s.step = stateError
s.err = &SyntaxError{"invalid character " + quoteChar(c) + " " + context, s.bytes}
return scanError
}
// quoteChar formats c as a quoted character literal
func quoteChar(c int) string {
// special cases - different from quoted strings
if c == '\'' {
return `'\''`
}
if c == '"' {
return `'"'`
}
// use quoted string with different quotation marks
s := strconv.Quote(string(c))
return "'" + s[1:len(s)-1] + "'"
}
// undo causes the scanner to return scanCode from the next state transition.
// This gives callers a simple 1-byte undo mechanism.
func (s *scanner) undo(scanCode int) {
if s.redo {
panic("json: invalid use of scanner")
}
s.redoCode = scanCode
s.redoState = s.step
s.step = stateRedo
s.redo = true
}
// stateRedo helps implement the scanner's 1-byte undo.
func stateRedo(s *scanner, c int) int {
s.redo = false
s.step = s.redoState
return s.redoCode
}
| {
"content_hash": "c79a410039465cdba6b5d9e3ca36f431",
"timestamp": "",
"source": "github",
"line_count": 619,
"max_line_length": 87,
"avg_line_length": 26.709208400646204,
"alnum_prop": 0.6652150244964616,
"repo_name": "connectordb/njson",
"id": "209d1a2bc2b482319181cc901fe82f4925658805",
"size": "16694",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "scanner.go",
"mode": "33188",
"license": "bsd-3-clause",
"language": [
{
"name": "Go",
"bytes": "158641"
}
],
"symlink_target": ""
} |
package com.google.android.glass.sample.kittycompass;
import com.google.android.glass.sample.kittycompass.model.Place;
import com.google.android.glass.sample.kittycompass.util.MathUtils;
import android.animation.Animator;
import android.animation.AnimatorListenerAdapter;
import android.animation.ValueAnimator;
import android.animation.ValueAnimator.AnimatorUpdateListener;
import android.content.Context;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.graphics.Canvas;
import android.graphics.Color;
import android.graphics.Paint;
import android.graphics.Path;
import android.graphics.Rect;
import android.graphics.Typeface;
import android.location.Location;
import android.text.TextPaint;
import android.util.AttributeSet;
import android.view.View;
import android.view.animation.LinearInterpolator;
import java.io.File;
import java.text.NumberFormat;
import java.util.ArrayList;
import java.util.List;
/**
* Draws a stylized compass, with text labels at the cardinal and ordinal directions, and tick
* marks at the half-winds. The red "needles" in the display mark the current heading.
*/
public class CompassView extends View {
/** Various dimensions and other drawing-related constants. */
private static final float NEEDLE_WIDTH = 6;
private static final float NEEDLE_HEIGHT = 125;
private static final int NEEDLE_COLOR = Color.RED;
private static final float TICK_WIDTH = 2;
private static final float TICK_HEIGHT = 10;
private static final float DIRECTION_TEXT_HEIGHT = 84.0f;
private static final float PLACE_TEXT_HEIGHT = 22.0f;
private static final float PLACE_PIN_WIDTH = 14.0f;
private static final float PLACE_TEXT_LEADING = 4.0f;
private static final float PLACE_TEXT_MARGIN = 8.0f;
/**
* The maximum number of places names to allow to stack vertically underneath the compass
* direction labels.
*/
private static final int MAX_OVERLAPPING_PLACE_NAMES = 4;
/**
* If the difference between two consecutive headings is less than this value, the canvas will
* be redrawn immediately rather than animated.
*/
private static final float MIN_DISTANCE_TO_ANIMATE = 15.0f;
/** The actual heading that represents the direction that the user is facing. */
private float mHeading;
/**
* Represents the heading that is currently being displayed when the view is drawn. This is
* used during animations, to keep track of the heading that should be drawn on the current
* frame, which may be different than the desired end point.
*/
private float mAnimatedHeading;
private OrientationManager mOrientation;
private List<Place> mNearbyPlaces;
private final Paint mPaint;
private final Paint mTickPaint;
private final Path mPath;
private final TextPaint mPlacePaint;
private final Bitmap mPlaceBitmap;
private final Rect mTextBounds;
private final List<Rect> mAllBounds;
private final NumberFormat mDistanceFormat;
private final String[] mDirections;
private final ValueAnimator mAnimator;
public CompassView(Context context) {
this(context, null, 0);
}
public CompassView(Context context, AttributeSet attrs) {
this(context, attrs, 0);
}
public CompassView(Context context, AttributeSet attrs, int defStyle) {
super(context, attrs, defStyle);
mPaint = new Paint();
mPaint.setStyle(Paint.Style.FILL);
mPaint.setAntiAlias(true);
mPaint.setTextSize(DIRECTION_TEXT_HEIGHT);
mPaint.setTypeface(Typeface.createFromFile(new File("/system/glass_fonts",
"Roboto-Thin.ttf")));
mTickPaint = new Paint();
mTickPaint.setStyle(Paint.Style.STROKE);
mTickPaint.setStrokeWidth(TICK_WIDTH);
mTickPaint.setAntiAlias(true);
mTickPaint.setColor(Color.WHITE);
mPlacePaint = new TextPaint();
mPlacePaint.setStyle(Paint.Style.FILL);
mPlacePaint.setAntiAlias(true);
mPlacePaint.setColor(Color.WHITE);
mPlacePaint.setTextSize(PLACE_TEXT_HEIGHT);
mPlacePaint.setTypeface(Typeface.createFromFile(new File("/system/glass_fonts",
"Roboto-Light.ttf")));
mPath = new Path();
mTextBounds = new Rect();
mAllBounds = new ArrayList<Rect>();
mDistanceFormat = NumberFormat.getNumberInstance();
mDistanceFormat.setMinimumFractionDigits(0);
mDistanceFormat.setMaximumFractionDigits(1);
mPlaceBitmap = BitmapFactory.decodeResource(context.getResources(), R.drawable.place_mark);
// We use NaN to indicate that the compass is being drawn for the first
// time, so that we can jump directly to the starting orientation
// instead of spinning from a default value of 0.
mAnimatedHeading = Float.NaN;
mDirections = context.getResources().getStringArray(R.array.direction_abbreviations);
mAnimator = new ValueAnimator();
setupAnimator();
}
/**
* Sets the instance of {@link OrientationManager} that this view will use to get the current
* heading and location.
*
* @param orientationManager the instance of {@code OrientationManager} that this view will use
*/
public void setOrientationManager(OrientationManager orientationManager) {
mOrientation = orientationManager;
}
/**
* Gets the current heading in degrees.
*
* @return the current heading.
*/
public float getHeading() {
return mHeading;
}
/**
* Sets the current heading in degrees and redraws the compass. If the angle is not between 0
* and 360, it is shifted to be in that range.
*
* @param degrees the current heading
*/
public void setHeading(float degrees) {
mHeading = MathUtils.mod(degrees, 360.0f);
animateTo(mHeading);
}
/**
* Sets the list of nearby places that the compass should display. This list is recalculated
* whenever the user's location changes, so that only locations within a certain distance will
* be displayed.
*
* @param places the list of {@code Place}s that should be displayed
*/
public void setNearbyPlaces(List<Place> places) {
mNearbyPlaces = places;
}
@Override
protected void onDraw(Canvas canvas) {
super.onDraw(canvas);
// The view displays 90 degrees across its width so that one 90 degree head rotation is
// equal to one full view cycle.
float pixelsPerDegree = getWidth() / 90.0f;
float centerX = getWidth() / 2.0f;
float centerY = getHeight() / 2.0f;
canvas.save();
canvas.translate(-mAnimatedHeading * pixelsPerDegree + centerX, centerY);
// In order to ensure that places on a boundary close to 0 or 360 get drawn correctly, we
// draw them three times; once to the left, once at the "true" bearing, and once to the
// right.
for (int i = -1; i <= 1; i++) {
drawPlaces(canvas, pixelsPerDegree, i * pixelsPerDegree * 360);
}
drawCompassDirections(canvas, pixelsPerDegree);
canvas.restore();
mPaint.setColor(NEEDLE_COLOR);
drawNeedle(canvas, false);
drawNeedle(canvas, true);
}
/**
* Draws the compass direction strings (N, NW, W, etc.).
*
* @param canvas the {@link Canvas} upon which to draw
* @param pixelsPerDegree the size, in pixels, of one degree step
*/
private void drawCompassDirections(Canvas canvas, float pixelsPerDegree) {
float degreesPerTick = 360.0f / mDirections.length;
mPaint.setColor(Color.WHITE);
// We draw two extra ticks/labels on each side of the view so that the
// full range is visible even when the heading is approximately 0.
for (int i = -2; i <= mDirections.length + 2; i++) {
if (MathUtils.mod(i, 2) == 0) {
// Draw a text label for the even indices.
String direction = mDirections[MathUtils.mod(i, mDirections.length)];
mPaint.getTextBounds(direction, 0, direction.length(), mTextBounds);
canvas.drawText(direction,
i * degreesPerTick * pixelsPerDegree - mTextBounds.width() / 2,
mTextBounds.height() / 2, mPaint);
} else {
// Draw a tick mark for the odd indices.
canvas.drawLine(i * degreesPerTick * pixelsPerDegree, -TICK_HEIGHT / 2, i
* degreesPerTick * pixelsPerDegree, TICK_HEIGHT / 2, mTickPaint);
}
}
}
/**
* Draws the pins and text labels for the nearby list of places.
*
* @param canvas the {@link Canvas} upon which to draw
* @param pixelsPerDegree the size, in pixels, of one degree step
* @param offset the number of pixels to translate the drawing operations by in the horizontal
* direction; used because place names are drawn three times to get proper wraparound
*/
private void drawPlaces(Canvas canvas, float pixelsPerDegree, float offset) {
if (mOrientation.hasLocation() && mNearbyPlaces != null) {
synchronized (mNearbyPlaces) {
Location userLocation = mOrientation.getLocation();
double latitude1 = userLocation.getLatitude();
double longitude1 = userLocation.getLongitude();
mAllBounds.clear();
// Loop over the list of nearby places (those within 10 km of the user's current
// location), and compute the relative bearing from the user's location to the
// place's location. This determines the position on the compass view where the
// pin will be drawn.
for (Place place : mNearbyPlaces) {
double latitude2 = place.getLatitude();
double longitude2 = place.getLongitude();
float bearing = MathUtils.getBearing(latitude1, longitude1, latitude2,
longitude2);
String name = place.getName();
double distanceKm = MathUtils.getDistance(latitude1, longitude1, latitude2,
longitude2);
String text = getContext().getResources().getString(
R.string.place_text_format, name, mDistanceFormat.format(distanceKm));
// Measure the text and offset the text bounds to the location where the text
// will finally be drawn.
Rect textBounds = new Rect();
mPlacePaint.getTextBounds(text, 0, text.length(), textBounds);
textBounds.offsetTo((int) (offset + bearing * pixelsPerDegree
+ PLACE_PIN_WIDTH / 2 + PLACE_TEXT_MARGIN), canvas.getHeight() / 2
- (int) PLACE_TEXT_HEIGHT);
// Extend the bounds rectangle to include the pin icon and a small margin
// to the right of the text, for the overlap calculations below.
textBounds.left -= PLACE_PIN_WIDTH + PLACE_TEXT_MARGIN;
textBounds.right += PLACE_TEXT_MARGIN;
// This loop attempts to find the best vertical position for the string by
// starting at the bottom of the display and checking to see if it overlaps
// with any other labels that were already drawn. If there is an overlap, we
// move up and check again, repeating this process until we find a vertical
// position where there is no overlap, or when we reach the limit on
// overlapping place names.
boolean intersects;
int numberOfTries = 0;
do {
intersects = false;
numberOfTries++;
textBounds.offset(0, (int) -(PLACE_TEXT_HEIGHT + PLACE_TEXT_LEADING));
for (Rect existing : mAllBounds) {
if (Rect.intersects(existing, textBounds)) {
intersects = true;
break;
}
}
} while (intersects && numberOfTries <= MAX_OVERLAPPING_PLACE_NAMES);
// Only draw the string if it would not go high enough to overlap the compass
// directions. This means some places may not be drawn, even if they're nearby.
if (numberOfTries <= MAX_OVERLAPPING_PLACE_NAMES) {
mAllBounds.add(textBounds);
canvas.drawBitmap(mPlaceBitmap, offset + bearing * pixelsPerDegree
- PLACE_PIN_WIDTH / 2, textBounds.top + 2, mPaint);
canvas.drawText(text,
offset + bearing * pixelsPerDegree + PLACE_PIN_WIDTH / 2
+ PLACE_TEXT_MARGIN, textBounds.top + PLACE_TEXT_HEIGHT,
mPlacePaint);
}
}
}
}
}
/**
* Draws a needle that is centered at the top or bottom of the compass.
*
* @param canvas the {@link Canvas} upon which to draw
* @param bottom true to draw the bottom needle, or false to draw the top needle
*/
private void drawNeedle(Canvas canvas, boolean bottom) {
float centerX = getWidth() / 2.0f;
float origin;
float sign;
// Flip the vertical coordinates if we're drawing the bottom needle.
if (bottom) {
origin = getHeight();
sign = -1;
} else {
origin = 0;
sign = 1;
}
float needleHalfWidth = NEEDLE_WIDTH / 2;
mPath.reset();
mPath.moveTo(centerX - needleHalfWidth, origin);
mPath.lineTo(centerX - needleHalfWidth, origin + sign * (NEEDLE_HEIGHT - 4));
mPath.lineTo(centerX, origin + sign * NEEDLE_HEIGHT);
mPath.lineTo(centerX + needleHalfWidth, origin + sign * (NEEDLE_HEIGHT - 4));
mPath.lineTo(centerX + needleHalfWidth, origin);
mPath.close();
canvas.drawPath(mPath, mPaint);
}
/**
* Sets up a {@link ValueAnimator} that will be used to animate the compass
* when the distance between two sensor events is large.
*/
private void setupAnimator() {
mAnimator.setInterpolator(new LinearInterpolator());
mAnimator.setDuration(250);
// Notifies us at each frame of the animation so we can redraw the view.
mAnimator.addUpdateListener(new AnimatorUpdateListener() {
@Override
public void onAnimationUpdate(ValueAnimator animator) {
mAnimatedHeading = MathUtils.mod((Float) mAnimator.getAnimatedValue(), 360.0f);
invalidate();
}
});
// Notifies us when the animation is over. During an animation, the user's head may have
// continued to move to a different orientation than the original destination angle of the
// animation. Since we can't easily change the animation goal while it is running, we call
// animateTo() again, which will either redraw at the new orientation (if the difference is
// small enough), or start another animation to the new heading. This seems to produce
// fluid results.
mAnimator.addListener(new AnimatorListenerAdapter() {
@Override
public void onAnimationEnd(Animator animator) {
animateTo(mHeading);
}
});
}
/**
* Animates the view to the specified heading, or simply redraws it immediately if the
* difference between the current heading and new heading are small enough that it wouldn't be
* noticeable.
*
* @param end the desired heading
*/
private void animateTo(float end) {
// Only act if the animator is not currently running. If the user's orientation changes
// while the animator is running, we wait until the end of the animation to update the
// display again, to prevent jerkiness.
if (!mAnimator.isRunning()) {
float start = mAnimatedHeading;
float distance = Math.abs(end - start);
float reverseDistance = 360.0f - distance;
float shortest = Math.min(distance, reverseDistance);
if (Float.isNaN(mAnimatedHeading) || shortest < MIN_DISTANCE_TO_ANIMATE) {
// If the distance to the destination angle is small enough (or if this is the
// first time the compass is being displayed), it will be more fluid to just redraw
// immediately instead of doing an animation.
mAnimatedHeading = end;
invalidate();
} else {
// For larger distances (i.e., if the compass "jumps" because of sensor calibration
// issues), we animate the effect to provide a more fluid user experience. The
// calculation below finds the shortest distance between the two angles, which may
// involve crossing 0/360 degrees.
float goal;
if (distance < reverseDistance) {
goal = end;
} else if (end < start) {
goal = end + 360.0f;
} else {
goal = end - 360.0f;
}
mAnimator.setFloatValues(start, goal);
mAnimator.start();
}
}
}
}
| {
"content_hash": "ee5a4a8245e85c3a6d5357b503400d35",
"timestamp": "",
"source": "github",
"line_count": 432,
"max_line_length": 99,
"avg_line_length": 41.46527777777778,
"alnum_prop": 0.615530620219952,
"repo_name": "mimming/kitty-compass",
"id": "d8f75fe28072be47697c6d74087217881ddadea6",
"size": "18507",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "gdk/src/com/google/android/glass/sample/kittycompass/CompassView.java",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "CSS",
"bytes": "358"
},
{
"name": "Java",
"bytes": "119106"
}
],
"symlink_target": ""
} |
{% capture question %}
Write a function in Scheme that rotates a list by k elements. Rotating a list by 1 consists of moving the first item to the end of the list. Rotating a list by k consists of moving the first k items to the end of the list. `k` can be any number (not constrained by the size of the list).
(define (rotate lst k)
; Your Code Here
)
And here's a test to help you out:
(define (test)
(assert-equal (rotate '(1 2 3 4) 3) '(4 1 2 3))
(assert-equal (rotate '(1 2) 3) '(2 1))
(assert-equal (rotate '(1 2 3 4 5) 10) '(1 2 3 4 5)))
{% endcapture %}
{% capture solution %}
(define (rotate lst k)
(cond ((null? lst) lst)
((= k 0) lst)
(else (rotate (append (cdr lst) (list (car lst))) (- k 1)))))
If `lst` is initially empty, then any input will return the same list (the empty list) so we should just go ahead and return it. If `k` is equal to zero, meaning that there are no more rotations, then return `lst`. Otherwise, we want to `rotate` the list while keeping all of the elements intact. That means appending the first item to the end of the rest of the list. We also want to make sure we decrement `k` by 1 meaning that we just successfully completed one rotation.
{% endcapture %}
{% include cs61a/problem_template.md %}
| {
"content_hash": "ea508b8fc0551f710e06bcfb4104c23f",
"timestamp": "",
"source": "github",
"line_count": 26,
"max_line_length": 474,
"avg_line_length": 52.34615384615385,
"alnum_prop": 0.6414401175606171,
"repo_name": "negativetwelve/markmiyashita.com",
"id": "53a0b0b263307c1935e44c6217d25d907e498e93",
"size": "1361",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "_includes/cs61a/problems/scheme/rotate_list_k_times.md",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "CSS",
"bytes": "25035"
},
{
"name": "Java",
"bytes": "954"
},
{
"name": "JavaScript",
"bytes": "743"
},
{
"name": "Python",
"bytes": "3492"
},
{
"name": "Ruby",
"bytes": "16279"
}
],
"symlink_target": ""
} |
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.messages = exports.ruleName = undefined;
exports.default = function (max) {
return function (root, result) {
var validOptions = (0, _utils.validateOptions)(result, ruleName, {
actual: max,
possible: [function (max) {
// Check that the max specificity is in the form "a,b,c"
var pattern = new RegExp("^\\d+,\\d+,\\d+$");
return pattern.test(max);
}]
});
if (!validOptions) {
return;
}
var maxSpecificityArray = ("0," + max).split(",").map(parseFloat);
root.walkRules(function (rule) {
if (!(0, _utils.isStandardSyntaxRule)(rule)) {
return;
}
if (!(0, _utils.isStandardSyntaxSelector)(rule.selector)) {
return;
}
// Using rule.selectors gets us each selector in the eventuality we have a comma separated set
rule.selectors.forEach(function (selector) {
(0, _postcssResolveNestedSelector2.default)(selector, rule).forEach(function (resolvedSelector) {
// Return early if selector contains a not pseudo-class
if (selector.indexOf(":not(") !== -1) {
return;
}
// Return early if selector contains a matches
if (selector.indexOf(":matches(") !== -1) {
return;
}
// Check if the selector specificity exceeds the allowed maximum
try {
if ((0, _specificity.compare)(resolvedSelector, maxSpecificityArray) === 1) {
(0, _utils.report)({
ruleName: ruleName,
result: result,
node: rule,
message: messages.expected(resolvedSelector, max),
word: selector
});
}
} catch (e) {
result.warn("Cannot parse selector", { node: rule });
}
});
});
});
};
};
var _utils = require("../../utils");
var _specificity = require("specificity");
var _postcssResolveNestedSelector = require("postcss-resolve-nested-selector");
var _postcssResolveNestedSelector2 = _interopRequireDefault(_postcssResolveNestedSelector);
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
var ruleName = exports.ruleName = "selector-max-specificity";
var messages = exports.messages = (0, _utils.ruleMessages)(ruleName, {
expected: function expected(selector, specificity) {
return "Expected \"" + selector + "\" to have a specificity no more than \"" + specificity + "\"";
}
}); | {
"content_hash": "640e5bb195219df504e9b9a8f681667f",
"timestamp": "",
"source": "github",
"line_count": 77,
"max_line_length": 105,
"avg_line_length": 33.753246753246756,
"alnum_prop": 0.5906117737591381,
"repo_name": "MattKraatz/MattKraatz.github.io",
"id": "c2407c6fd8ad2070a1edccace13fd6617d8953e1",
"size": "2599",
"binary": false,
"copies": "7",
"ref": "refs/heads/master",
"path": "_site/node_modules/stylelint/dist/rules/selector-max-specificity/index.js",
"mode": "33261",
"license": "mit",
"language": [
{
"name": "CSS",
"bytes": "9020"
},
{
"name": "HTML",
"bytes": "104902"
},
{
"name": "JavaScript",
"bytes": "7746"
}
],
"symlink_target": ""
} |
#ifndef ZDP_H
#define ZDP_H
#include <cstdint>
namespace deCONZ
{
class Address;
class ApsController;
class Binding;
}
class ZDP_Binding
{
public:
uint64_t srcExtAddress;
bool isValid() const { return (isUnicastBinding || isGroupBinding) && srcEndpoint != 0; }
union
{
uint16_t dstGroup;
uint64_t dstExtAddress;
};
uint16_t clusterId;
uint8_t srcEndpoint;
uint8_t dstEndpoint;
struct
{
unsigned int isGroupBinding : 1;
unsigned int isUnicastBinding : 1;
unsigned int pad : 6 + 24;
};
};
struct ZDP_Result
{
bool isEnqueued = false; //! true when request was accepted for the APS request queue.
uint8_t apsReqId = 0; //! Underlying deCONZ::ApsDataRequest::id() to match in confirm.
uint8_t zdpSeq = 0; //! ZDP sequence number.
/*! To check ZDP_Result in an if statement: e.g. `if(ZDP_SomeReq()) ...` */
operator bool() const
{
return isEnqueued;
}
};
ZDP_Result ZDP_NodeDescriptorReq(const deCONZ::Address &addr, deCONZ::ApsController *apsCtrl);
ZDP_Result ZDP_ActiveEndpointsReq(const deCONZ::Address &addr, deCONZ::ApsController *apsCtrl);
ZDP_Result ZDP_SimpleDescriptorReq(const deCONZ::Address &addr, uint8_t endpoint, deCONZ::ApsController *apsCtrl);
ZDP_Result ZDP_BindReq(const deCONZ::Binding &bnd, deCONZ::ApsController *apsCtrl);
ZDP_Result ZDP_UnbindReq(const deCONZ::Binding &bnd, deCONZ::ApsController *apsCtrl);
ZDP_Result ZDP_MgmtBindReq(uint8_t startIndex, const deCONZ::Address &addr, deCONZ::ApsController *apsCtrl);
uint8_t ZDP_NextSequenceNumber();
#endif // ZDP_H
| {
"content_hash": "df776091dff2bc4cf56182c5ab4a1897",
"timestamp": "",
"source": "github",
"line_count": 59,
"max_line_length": 114,
"avg_line_length": 27.728813559322035,
"alnum_prop": 0.684596577017115,
"repo_name": "dresden-elektronik/deconz-rest-plugin",
"id": "48c46df68299929658167062056c8f7a5c256754",
"size": "1910",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "zdp/zdp.h",
"mode": "33188",
"license": "bsd-3-clause",
"language": [
{
"name": "C",
"bytes": "3897918"
},
{
"name": "C++",
"bytes": "3776495"
},
{
"name": "CMake",
"bytes": "4723"
},
{
"name": "JavaScript",
"bytes": "7069"
},
{
"name": "Python",
"bytes": "782"
},
{
"name": "QMake",
"bytes": "8765"
},
{
"name": "Shell",
"bytes": "29528"
}
],
"symlink_target": ""
} |
#pragma once
#include <QtCore/QThread>
#include <QtScript/QScriptEngine>
namespace trikScriptRunner {
class Threading;
/// Thread object which executes a script with a QScriptEngine
class ScriptThread : public QThread
{
Q_OBJECT
public:
/// Constructor
/// @param threading - threading manager for this thread
/// @param engine - QScriptEngine which will do the work
/// @param script - a Qt Script to run
ScriptThread(Threading &threading, const QString &id, QScriptEngine *engine, const QString &script);
~ScriptThread() override;
/// Aborts execution. Asynchronous: a thread will not be finished on return from this function
void abort();
/// @returns string id of the thread
QString id() const;
/// @returns error message or empty string if evalutation succeed
QString error() const;
/// @returns true if the script engine is evaluating a script at the moment
bool isEvaluating() const;
signals:
/// Emitted when event loop must be stopped.
void stopRunning();
protected:
void run() override;
private:
QString mId;
/// Has ownership (thru deleteLater() call).
QScriptEngine *mEngine;
QString mScript;
Threading &mThreading;
QString mError;
};
}
| {
"content_hash": "aba09fbad48ba4264b3e0ed920283886",
"timestamp": "",
"source": "github",
"line_count": 57,
"max_line_length": 101,
"avg_line_length": 20.912280701754387,
"alnum_prop": 0.735738255033557,
"repo_name": "vrxfile/trikRuntime",
"id": "1009e222f3eecee07b19d6028453ac22a3f63585",
"size": "1790",
"binary": false,
"copies": "4",
"ref": "refs/heads/master",
"path": "trikScriptRunner/src/scriptThread.h",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "Batchfile",
"bytes": "87"
},
{
"name": "C",
"bytes": "3852"
},
{
"name": "C++",
"bytes": "797997"
},
{
"name": "JavaScript",
"bytes": "4537"
},
{
"name": "Prolog",
"bytes": "1188"
},
{
"name": "QMake",
"bytes": "35762"
},
{
"name": "Shell",
"bytes": "256"
},
{
"name": "Tcl",
"bytes": "20341"
}
],
"symlink_target": ""
} |
<!doctype html>
<html class="default no-js">
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<title>QueryDelete | ng-db-helper</title>
<meta name="description" content="">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="../assets/css/main.css">
</head>
<body>
<header>
<div class="tsd-page-toolbar">
<div class="container">
<div class="table-wrap">
<div class="table-cell" id="tsd-search" data-index="../assets/js/search.js" data-base="..">
<div class="field">
<label for="tsd-search-field" class="tsd-widget search no-caption">Search</label>
<input id="tsd-search-field" type="text" />
</div>
<ul class="results">
<li class="state loading">Preparing search index...</li>
<li class="state failure">The search index is not available</li>
</ul>
<a href="../index.html" class="title">ng-db-helper</a>
</div>
<div class="table-cell" id="tsd-widgets">
<div id="tsd-filter">
<a href="#" class="tsd-widget options no-caption" data-toggle="options">Options</a>
<div class="tsd-filter-group">
<div class="tsd-select" id="tsd-filter-visibility">
<span class="tsd-select-label">All</span>
<ul class="tsd-select-list">
<li data-value="public">Public</li>
<li data-value="protected">Public/Protected</li>
<li data-value="private" class="selected">All</li>
</ul>
</div>
<input type="checkbox" id="tsd-filter-inherited" checked />
<label class="tsd-widget" for="tsd-filter-inherited">Inherited</label>
<input type="checkbox" id="tsd-filter-externals" checked />
<label class="tsd-widget" for="tsd-filter-externals">Externals</label>
<input type="checkbox" id="tsd-filter-only-exported" />
<label class="tsd-widget" for="tsd-filter-only-exported">Only exported</label>
</div>
</div>
<a href="#" class="tsd-widget menu no-caption" data-toggle="menu">Menu</a>
</div>
</div>
</div>
</div>
<div class="tsd-page-title">
<div class="container">
<ul class="tsd-breadcrumb">
<li>
<a href="../globals.html">Globals</a>
</li>
<li>
<a href="querydelete.html">QueryDelete</a>
</li>
</ul>
<h1>Class QueryDelete<T></h1>
</div>
</div>
</header>
<div class="container container-main">
<div class="row">
<div class="col-8 col-content">
<section class="tsd-panel tsd-comment">
<div class="tsd-comment tsd-typography">
<dl class="tsd-comment-tags">
<dt>class</dt>
<dd><p>QueryDelete</p>
</dd>
<dt>description</dt>
<dd><p>For design reasons this class should not be used directly. Use this class through <a href="../globals.html#delete">Delete</a> function.</p>
</dd>
<dt>example</dt>
<dd><pre><code class="lang-typesrcript">// Delete a specific model using Delete
// assume that "todo" is declared before and is a model extending
// DbHelperModel and using Table + Column annotation
Delete(todo).exec().subscribe((qr: QueryResult<any>) => {
// the model is deleted...
}, (err) => {
// manage th error...
});
// You could use Delete statement to delete many entries
Delete(Todo).where({isDone: true}).exec().subscribe((qr: QueryResult<any>) => {
// all done todos are deleted !
}, (err) => {
// manage th error...
});
</code></pre>
</dd>
<dt>author</dt>
<dd><p>Olivier Margarit</p>
</dd>
<dt>since</dt>
<dd><p>0.1</p>
</dd>
</dl>
</div>
</section>
<section class="tsd-panel tsd-type-parameters">
<h3>Type parameters</h3>
<ul class="tsd-type-parameters">
<li>
<h4>T<span class="tsd-signature-symbol">: </span><a href="dbhelpermodel.html" class="tsd-signature-type">DbHelperModel</a></h4>
<div class="tsd-comment tsd-typography">
<div class="lead">
<p>@extends DbHelperModel a model declared with table
and column annotations</p>
</div>
</div>
</li>
</ul>
</section>
<section class="tsd-panel tsd-hierarchy">
<h3>Hierarchy</h3>
<ul class="tsd-hierarchy">
<li>
<span class="target">QueryDelete</span>
</li>
</ul>
</section>
<section class="tsd-panel-group tsd-index-group">
<h2>Index</h2>
<section class="tsd-panel tsd-index-panel">
<div class="tsd-index-content">
<section class="tsd-index-section tsd-is-external">
<h3>Constructors</h3>
<ul class="tsd-index-list">
<li class="tsd-kind-constructor tsd-parent-kind-class tsd-is-external"><a href="querydelete.html#constructor" class="tsd-kind-icon">constructor</a></li>
</ul>
</section>
<section class="tsd-index-section tsd-is-private tsd-is-private-protected tsd-is-external">
<h3>Properties</h3>
<ul class="tsd-index-list">
<li class="tsd-kind-property tsd-parent-kind-class tsd-is-private tsd-is-external"><a href="querydelete.html#model" class="tsd-kind-icon">model</a></li>
<li class="tsd-kind-property tsd-parent-kind-class tsd-is-private tsd-is-external"><a href="querydelete.html#type" class="tsd-kind-icon">type</a></li>
<li class="tsd-kind-property tsd-parent-kind-class tsd-is-private tsd-is-external"><a href="querydelete.html#whereclauses" class="tsd-kind-icon">where<wbr>Clauses</a></li>
</ul>
</section>
<section class="tsd-index-section tsd-is-external">
<h3>Methods</h3>
<ul class="tsd-index-list">
<li class="tsd-kind-method tsd-parent-kind-class tsd-is-external"><a href="querydelete.html#build" class="tsd-kind-icon">build</a></li>
<li class="tsd-kind-method tsd-parent-kind-class tsd-is-external"><a href="querydelete.html#exec" class="tsd-kind-icon">exec</a></li>
<li class="tsd-kind-method tsd-parent-kind-class tsd-is-external"><a href="querydelete.html#where" class="tsd-kind-icon">where</a></li>
</ul>
</section>
</div>
</section>
</section>
<section class="tsd-panel-group tsd-member-group tsd-is-external">
<h2>Constructors</h2>
<section class="tsd-panel tsd-member tsd-kind-constructor tsd-parent-kind-class tsd-is-external">
<a name="constructor" class="tsd-anchor"></a>
<h3>constructor</h3>
<ul class="tsd-signatures tsd-kind-constructor tsd-parent-kind-class tsd-is-external">
<li class="tsd-signature tsd-kind-icon">new <wbr>Query<wbr>Delete<span class="tsd-signature-symbol">(</span>model<span class="tsd-signature-symbol">: </span><span class="tsd-signature-type">T</span><span class="tsd-signature-symbol"> | </span><span class="tsd-signature-type">object</span><span class="tsd-signature-symbol">)</span><span class="tsd-signature-symbol">: </span><a href="querydelete.html" class="tsd-signature-type">QueryDelete</a></li>
</ul>
<ul class="tsd-descriptions">
<li class="tsd-description">
<aside class="tsd-sources">
<ul>
<li>Defined in <a href="https://github.com/margarito/ng-db-helper/blob/e02f13c/lib/core/models/queries/delete.model.ts#L56">lib/core/models/queries/delete.model.ts:56</a></li>
</ul>
</aside>
<div class="tsd-comment tsd-typography">
<dl class="tsd-comment-tags">
<dt>constructor</dt>
<dd><p>should not be use directly, see class header</p>
</dd>
</dl>
</div>
<h4 class="tsd-parameters-title">Parameters</h4>
<ul class="tsd-parameters">
<li>
<h5>model: <span class="tsd-signature-type">T</span><span class="tsd-signature-symbol"> | </span><span class="tsd-signature-type">object</span></h5>
<div class="tsd-comment tsd-typography">
<div class="lead">
<p>DbHelperModel extention</p>
</div>
</div>
</li>
</ul>
<h4 class="tsd-returns-title">Returns <a href="querydelete.html" class="tsd-signature-type">QueryDelete</a></h4>
</li>
</ul>
</section>
</section>
<section class="tsd-panel-group tsd-member-group tsd-is-private tsd-is-private-protected tsd-is-external">
<h2>Properties</h2>
<section class="tsd-panel tsd-member tsd-kind-property tsd-parent-kind-class tsd-is-private tsd-is-external">
<a name="model" class="tsd-anchor"></a>
<h3><span class="tsd-flag ts-flagPrivate">Private</span> model</h3>
<div class="tsd-signature tsd-kind-icon">model<span class="tsd-signature-symbol">:</span> <span class="tsd-signature-type">T</span><span class="tsd-signature-symbol"> | </span><span class="tsd-signature-type">object</span></div>
<aside class="tsd-sources">
<ul>
<li>Defined in <a href="https://github.com/margarito/ng-db-helper/blob/e02f13c/lib/core/models/queries/delete.model.ts#L64">lib/core/models/queries/delete.model.ts:64</a></li>
</ul>
</aside>
<div class="tsd-comment tsd-typography">
<div class="lead">
<p>DbHelperModel extention</p>
</div>
</div>
</section>
<section class="tsd-panel tsd-member tsd-kind-property tsd-parent-kind-class tsd-is-private tsd-is-external">
<a name="type" class="tsd-anchor"></a>
<h3><span class="tsd-flag ts-flagPrivate">Private</span> type</h3>
<div class="tsd-signature tsd-kind-icon">type<span class="tsd-signature-symbol">:</span> <span class="tsd-signature-type">"DELETE"</span><span class="tsd-signature-symbol"> = "DELETE"</span></div>
<aside class="tsd-sources">
<ul>
<li>Defined in <a href="https://github.com/margarito/ng-db-helper/blob/e02f13c/lib/core/models/queries/delete.model.ts#L49">lib/core/models/queries/delete.model.ts:49</a></li>
</ul>
</aside>
<div class="tsd-comment tsd-typography">
<dl class="tsd-comment-tags">
<dt>constant</dt>
<dd><p>{string} type the type of statement, should not be modified</p>
</dd>
</dl>
</div>
</section>
<section class="tsd-panel tsd-member tsd-kind-property tsd-parent-kind-class tsd-is-private tsd-is-external">
<a name="whereclauses" class="tsd-anchor"></a>
<h3><span class="tsd-flag ts-flagPrivate">Private</span> where<wbr>Clauses</h3>
<div class="tsd-signature tsd-kind-icon">where<wbr>Clauses<span class="tsd-signature-symbol">:</span> <a href="clausegroup.html" class="tsd-signature-type">ClauseGroup</a></div>
<aside class="tsd-sources">
<ul>
<li>Defined in <a href="https://github.com/margarito/ng-db-helper/blob/e02f13c/lib/core/models/queries/delete.model.ts#L56">lib/core/models/queries/delete.model.ts:56</a></li>
</ul>
</aside>
<div class="tsd-comment tsd-typography">
<dl class="tsd-comment-tags">
<dt>property</dt>
<dd><p>{ClauseGroup} whereClauses is <a href="clausegroup.html">ClauseGroup</a> instance containing
where clauses</p>
</dd>
</dl>
</div>
</section>
</section>
<section class="tsd-panel-group tsd-member-group tsd-is-external">
<h2>Methods</h2>
<section class="tsd-panel tsd-member tsd-kind-method tsd-parent-kind-class tsd-is-external">
<a name="build" class="tsd-anchor"></a>
<h3>build</h3>
<ul class="tsd-signatures tsd-kind-method tsd-parent-kind-class tsd-is-external">
<li class="tsd-signature tsd-kind-icon">build<span class="tsd-signature-symbol">(</span><span class="tsd-signature-symbol">)</span><span class="tsd-signature-symbol">: </span><a href="dbquery.html" class="tsd-signature-type">DbQuery</a></li>
</ul>
<ul class="tsd-descriptions">
<li class="tsd-description">
<aside class="tsd-sources">
<ul>
<li>Defined in <a href="https://github.com/margarito/ng-db-helper/blob/e02f13c/lib/core/models/queries/delete.model.ts#L90">lib/core/models/queries/delete.model.ts:90</a></li>
</ul>
</aside>
<div class="tsd-comment tsd-typography">
<dl class="tsd-comment-tags">
<dt>method</dt>
<dd><p>build should be removed to be a part of the private API</p>
</dd>
</dl>
</div>
<h4 class="tsd-returns-title">Returns <a href="dbquery.html" class="tsd-signature-type">DbQuery</a></h4>
<p>of the query with the string part and
clauses params.</p>
</li>
</ul>
</section>
<section class="tsd-panel tsd-member tsd-kind-method tsd-parent-kind-class tsd-is-external">
<a name="exec" class="tsd-anchor"></a>
<h3>exec</h3>
<ul class="tsd-signatures tsd-kind-method tsd-parent-kind-class tsd-is-external">
<li class="tsd-signature tsd-kind-icon">exec<span class="tsd-signature-symbol">(</span><span class="tsd-signature-symbol">)</span><span class="tsd-signature-symbol">: </span><span class="tsd-signature-type">Observable</span><span class="tsd-signature-symbol"><</span><a href="../interfaces/queryresult.html" class="tsd-signature-type">QueryResult</a><span class="tsd-signature-symbol"><</span><span class="tsd-signature-type">any</span><span class="tsd-signature-symbol">></span><span class="tsd-signature-symbol">></span></li>
</ul>
<ul class="tsd-descriptions">
<li class="tsd-description">
<aside class="tsd-sources">
<ul>
<li>Defined in <a href="https://github.com/margarito/ng-db-helper/blob/e02f13c/lib/core/models/queries/delete.model.ts#L120">lib/core/models/queries/delete.model.ts:120</a></li>
</ul>
</aside>
<div class="tsd-comment tsd-typography">
<dl class="tsd-comment-tags">
<dt>method</dt>
<dd><p>exec to execute the query and asynchronously retreive result.</p>
</dd>
</dl>
</div>
<h4 class="tsd-returns-title">Returns <span class="tsd-signature-type">Observable</span><span class="tsd-signature-symbol"><</span><a href="../interfaces/queryresult.html" class="tsd-signature-type">QueryResult</a><span class="tsd-signature-symbol"><</span><span class="tsd-signature-type">any</span><span class="tsd-signature-symbol">></span><span class="tsd-signature-symbol">></span></h4>
<p>observable to subscribe and retrieve results</p>
</li>
</ul>
</section>
<section class="tsd-panel tsd-member tsd-kind-method tsd-parent-kind-class tsd-is-external">
<a name="where" class="tsd-anchor"></a>
<h3>where</h3>
<ul class="tsd-signatures tsd-kind-method tsd-parent-kind-class tsd-is-external">
<li class="tsd-signature tsd-kind-icon">where<span class="tsd-signature-symbol">(</span>clauses<span class="tsd-signature-symbol">: </span><a href="clause.html" class="tsd-signature-type">Clause</a><span class="tsd-signature-symbol"> | </span><a href="clause.html" class="tsd-signature-type">Clause</a><span class="tsd-signature-symbol">[]</span><span class="tsd-signature-symbol"> | </span><a href="clausegroup.html" class="tsd-signature-type">ClauseGroup</a><span class="tsd-signature-symbol"> | </span><a href="compositeclause.html" class="tsd-signature-type">CompositeClause</a><span class="tsd-signature-symbol"> | </span><span class="tsd-signature-type">object</span><span class="tsd-signature-symbol">)</span><span class="tsd-signature-symbol">: </span><a href="querydelete.html" class="tsd-signature-type">QueryDelete</a><span class="tsd-signature-symbol"><</span><span class="tsd-signature-type">T</span><span class="tsd-signature-symbol">></span></li>
</ul>
<ul class="tsd-descriptions">
<li class="tsd-description">
<aside class="tsd-sources">
<ul>
<li>Defined in <a href="https://github.com/margarito/ng-db-helper/blob/e02f13c/lib/core/models/queries/delete.model.ts#L75">lib/core/models/queries/delete.model.ts:75</a></li>
</ul>
</aside>
<div class="tsd-comment tsd-typography">
<dl class="tsd-comment-tags">
<dt>method</dt>
<dd><p>where is the method to add clauses to the where statement of the query
see <a href="clause.html">Clause</a> or <a href="clausegroup.html">ClauseGroup</a></p>
</dd>
</dl>
</div>
<h4 class="tsd-parameters-title">Parameters</h4>
<ul class="tsd-parameters">
<li>
<h5>clauses: <a href="clause.html" class="tsd-signature-type">Clause</a><span class="tsd-signature-symbol"> | </span><a href="clause.html" class="tsd-signature-type">Clause</a><span class="tsd-signature-symbol">[]</span><span class="tsd-signature-symbol"> | </span><a href="clausegroup.html" class="tsd-signature-type">ClauseGroup</a><span class="tsd-signature-symbol"> | </span><a href="compositeclause.html" class="tsd-signature-type">CompositeClause</a><span class="tsd-signature-symbol"> | </span><span class="tsd-signature-type">object</span></h5>
<div class="tsd-comment tsd-typography">
<p>list of clauses</p>
</div>
</li>
</ul>
<h4 class="tsd-returns-title">Returns <a href="querydelete.html" class="tsd-signature-type">QueryDelete</a><span class="tsd-signature-symbol"><</span><span class="tsd-signature-type">T</span><span class="tsd-signature-symbol">></span></h4>
<p>this instance to chain query instructions</p>
</li>
</ul>
</section>
</section>
</div>
<div class="col-4 col-menu menu-sticky-wrap menu-highlight">
<nav class="tsd-navigation primary">
<ul>
<li class="globals ">
<a href="../globals.html"><em>Globals</em></a>
</li>
</ul>
</nav>
<nav class="tsd-navigation secondary menu-sticky">
<ul class="before-current">
</ul>
<ul class="current">
<li class="current tsd-kind-class tsd-has-type-parameter tsd-is-external">
<a href="querydelete.html" class="tsd-kind-icon">Query<wbr>Delete</a>
<ul>
<li class=" tsd-kind-constructor tsd-parent-kind-class tsd-is-external">
<a href="querydelete.html#constructor" class="tsd-kind-icon">constructor</a>
</li>
<li class=" tsd-kind-property tsd-parent-kind-class tsd-is-private tsd-is-external">
<a href="querydelete.html#model" class="tsd-kind-icon">model</a>
</li>
<li class=" tsd-kind-property tsd-parent-kind-class tsd-is-private tsd-is-external">
<a href="querydelete.html#type" class="tsd-kind-icon">type</a>
</li>
<li class=" tsd-kind-property tsd-parent-kind-class tsd-is-private tsd-is-external">
<a href="querydelete.html#whereclauses" class="tsd-kind-icon">where<wbr>Clauses</a>
</li>
<li class=" tsd-kind-method tsd-parent-kind-class tsd-is-external">
<a href="querydelete.html#build" class="tsd-kind-icon">build</a>
</li>
<li class=" tsd-kind-method tsd-parent-kind-class tsd-is-external">
<a href="querydelete.html#exec" class="tsd-kind-icon">exec</a>
</li>
<li class=" tsd-kind-method tsd-parent-kind-class tsd-is-external">
<a href="querydelete.html#where" class="tsd-kind-icon">where</a>
</li>
</ul>
</li>
</ul>
<ul class="after-current">
</ul>
</nav>
</div>
</div>
</div>
<footer class="with-border-bottom">
<div class="container">
<h2>Legend</h2>
<div class="tsd-legend-group">
<ul class="tsd-legend">
<li class="tsd-kind-module"><span class="tsd-kind-icon">Module</span></li>
<li class="tsd-kind-object-literal"><span class="tsd-kind-icon">Object literal</span></li>
<li class="tsd-kind-variable"><span class="tsd-kind-icon">Variable</span></li>
<li class="tsd-kind-function"><span class="tsd-kind-icon">Function</span></li>
<li class="tsd-kind-function tsd-has-type-parameter"><span class="tsd-kind-icon">Function with type parameter</span></li>
<li class="tsd-kind-index-signature"><span class="tsd-kind-icon">Index signature</span></li>
<li class="tsd-kind-type-alias"><span class="tsd-kind-icon">Type alias</span></li>
</ul>
<ul class="tsd-legend">
<li class="tsd-kind-enum"><span class="tsd-kind-icon">Enumeration</span></li>
<li class="tsd-kind-enum-member"><span class="tsd-kind-icon">Enumeration member</span></li>
<li class="tsd-kind-property tsd-parent-kind-enum"><span class="tsd-kind-icon">Property</span></li>
<li class="tsd-kind-method tsd-parent-kind-enum"><span class="tsd-kind-icon">Method</span></li>
</ul>
<ul class="tsd-legend">
<li class="tsd-kind-interface"><span class="tsd-kind-icon">Interface</span></li>
<li class="tsd-kind-interface tsd-has-type-parameter"><span class="tsd-kind-icon">Interface with type parameter</span></li>
<li class="tsd-kind-constructor tsd-parent-kind-interface"><span class="tsd-kind-icon">Constructor</span></li>
<li class="tsd-kind-property tsd-parent-kind-interface"><span class="tsd-kind-icon">Property</span></li>
<li class="tsd-kind-method tsd-parent-kind-interface"><span class="tsd-kind-icon">Method</span></li>
<li class="tsd-kind-index-signature tsd-parent-kind-interface"><span class="tsd-kind-icon">Index signature</span></li>
</ul>
<ul class="tsd-legend">
<li class="tsd-kind-class"><span class="tsd-kind-icon">Class</span></li>
<li class="tsd-kind-class tsd-has-type-parameter"><span class="tsd-kind-icon">Class with type parameter</span></li>
<li class="tsd-kind-constructor tsd-parent-kind-class"><span class="tsd-kind-icon">Constructor</span></li>
<li class="tsd-kind-property tsd-parent-kind-class"><span class="tsd-kind-icon">Property</span></li>
<li class="tsd-kind-method tsd-parent-kind-class"><span class="tsd-kind-icon">Method</span></li>
<li class="tsd-kind-accessor tsd-parent-kind-class"><span class="tsd-kind-icon">Accessor</span></li>
<li class="tsd-kind-index-signature tsd-parent-kind-class"><span class="tsd-kind-icon">Index signature</span></li>
</ul>
<ul class="tsd-legend">
<li class="tsd-kind-constructor tsd-parent-kind-class tsd-is-inherited"><span class="tsd-kind-icon">Inherited constructor</span></li>
<li class="tsd-kind-property tsd-parent-kind-class tsd-is-inherited"><span class="tsd-kind-icon">Inherited property</span></li>
<li class="tsd-kind-method tsd-parent-kind-class tsd-is-inherited"><span class="tsd-kind-icon">Inherited method</span></li>
<li class="tsd-kind-accessor tsd-parent-kind-class tsd-is-inherited"><span class="tsd-kind-icon">Inherited accessor</span></li>
</ul>
<ul class="tsd-legend">
<li class="tsd-kind-property tsd-parent-kind-class tsd-is-protected"><span class="tsd-kind-icon">Protected property</span></li>
<li class="tsd-kind-method tsd-parent-kind-class tsd-is-protected"><span class="tsd-kind-icon">Protected method</span></li>
<li class="tsd-kind-accessor tsd-parent-kind-class tsd-is-protected"><span class="tsd-kind-icon">Protected accessor</span></li>
</ul>
<ul class="tsd-legend">
<li class="tsd-kind-property tsd-parent-kind-class tsd-is-private"><span class="tsd-kind-icon">Private property</span></li>
<li class="tsd-kind-method tsd-parent-kind-class tsd-is-private"><span class="tsd-kind-icon">Private method</span></li>
<li class="tsd-kind-accessor tsd-parent-kind-class tsd-is-private"><span class="tsd-kind-icon">Private accessor</span></li>
</ul>
<ul class="tsd-legend">
<li class="tsd-kind-property tsd-parent-kind-class tsd-is-static"><span class="tsd-kind-icon">Static property</span></li>
<li class="tsd-kind-call-signature tsd-parent-kind-class tsd-is-static"><span class="tsd-kind-icon">Static method</span></li>
</ul>
</div>
</div>
</footer>
<div class="container tsd-generator">
<p>Generated using <a href="http://typedoc.org/" target="_blank">TypeDoc</a></p>
</div>
<div class="overlay"></div>
<script src="../assets/js/main.js"></script>
<script>if (location.protocol == 'file:') document.write('<script src="../assets/js/search.js"><' + '/script>');</script>
</body>
</html> | {
"content_hash": "6b89e12658231c6ef6fa26d4c4772fc8",
"timestamp": "",
"source": "github",
"line_count": 447,
"max_line_length": 973,
"avg_line_length": 53.608501118568235,
"alnum_prop": 0.6483328464716438,
"repo_name": "margarito/ng-db-helper",
"id": "dd3d3e08eb7cf334949e40f0187e9d19597e4300",
"size": "23963",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "documentation/classes/querydelete.html",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "JavaScript",
"bytes": "3768"
},
{
"name": "TypeScript",
"bytes": "52247"
}
],
"symlink_target": ""
} |
<mods xmlns="http://www.loc.gov/mods/v3" xmlns:exslt="http://exslt.org/common" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xlink="http://www.w3.org/1999/xlink" xsi:schemaLocation="http://www.loc.gov/mods/v3 http://www.loc.gov/standards/mods/v3/mods-3-3.xsd" version="3.3" ID="P0b002ee180e5d63d">
<name type="corporate">
<namePart>United States Government Printing Office</namePart>
<role>
<roleTerm type="text" authority="marcrelator">printer</roleTerm>
<roleTerm type="code" authority="marcrelator">prt</roleTerm>
</role>
<role>
<roleTerm type="text" authority="marcrelator">distributor</roleTerm>
<roleTerm type="code" authority="marcrelator">dst</roleTerm>
</role>
</name>
<name type="corporate">
<namePart>United States</namePart>
<namePart>United States Court of Appeals for the Eighth Circuit</namePart>
<role>
<roleTerm type="text" authority="marcrelator">author</roleTerm>
<roleTerm type="code" authority="marcrelator">aut</roleTerm>
</role>
<description>Government Organization</description>
</name>
<typeOfResource>text</typeOfResource>
<genre authority="marcgt">government publication</genre>
<language>
<languageTerm authority="iso639-2b" type="code">eng</languageTerm>
</language>
<extension>
<collectionCode>USCOURTS</collectionCode>
<category>Judicial Publications</category>
<branch>judicial</branch>
<dateIngested>2011-10-13</dateIngested>
</extension>
<originInfo>
<publisher>Administrative Office of the United States Courts</publisher>
<dateIssued encoding="w3cdtf">2007-01-04</dateIssued>
<issuance>monographic</issuance>
</originInfo>
<physicalDescription>
<note type="source content type">deposited</note>
<digitalOrigin>born digital</digitalOrigin>
</physicalDescription>
<classification authority="sudocs">JU 2.11</classification>
<identifier type="uri">http://www.gpo.gov/fdsys/pkg/USCOURTS-ca8-06-01807</identifier>
<identifier type="local">P0b002ee180e5d63d</identifier>
<recordInfo>
<recordContentSource authority="marcorg">DGPO</recordContentSource>
<recordCreationDate encoding="w3cdtf">2011-10-13</recordCreationDate>
<recordChangeDate encoding="w3cdtf">2011-10-13</recordChangeDate>
<recordIdentifier source="DGPO">USCOURTS-ca8-06-01807</recordIdentifier>
<recordOrigin>machine generated</recordOrigin>
<languageOfCataloging>
<languageTerm authority="iso639-2b" type="code">eng</languageTerm>
</languageOfCataloging>
</recordInfo>
<accessCondition type="GPO scope determination">fdlp</accessCondition>
<extension>
<docClass>USCOURTS</docClass>
<accessId>USCOURTS-ca8-06-01807</accessId>
<courtType>Appellate</courtType>
<courtCode>ca8</courtCode>
<courtCircuit>8th</courtCircuit>
<courtSortOrder>1080</courtSortOrder>
<caseNumber>06-01807</caseNumber>
<party firstName="Sylvester" fullName="Sylvester Richards Gayekpar" lastName="Gayekpar" middleName="Richards" role="Appellee"></party>
<party fullName="United States of America" lastName="United States of America" role="Appellant"></party>
</extension>
<titleInfo>
<title>United States v. Sylvester Gayekpar</title>
<partNumber>06-01807</partNumber>
</titleInfo>
<location>
<url access="object in context" displayLabel="Content Detail">http://www.gpo.gov/fdsys/pkg/USCOURTS-ca8-06-01807/content-detail.html</url>
</location>
<classification authority="sudocs">JU 2.11</classification>
<identifier type="preferred citation">06-01807;06-1807</identifier>
<name type="corporate">
<namePart>United States Court of Appeals for the Eighth Circuit</namePart>
<namePart>8th Circuit</namePart>
<namePart></namePart>
<affiliation>U.S. Courts</affiliation>
<role>
<roleTerm authority="marcrelator" type="text">author</roleTerm>
<roleTerm authority="marcrelator" type="code">aut</roleTerm>
</role>
</name>
<name type="personal">
<displayForm>Sylvester Richards Gayekpar</displayForm>
<namePart type="family">Gayekpar</namePart>
<namePart type="given">Sylvester</namePart>
<namePart type="termsOfAddress"></namePart>
<description>Appellee</description>
</name>
<name type="personal">
<displayForm>United States of America</displayForm>
<namePart type="family">United States of America</namePart>
<namePart type="given"></namePart>
<namePart type="termsOfAddress"></namePart>
<description>Appellant</description>
</name>
<extension>
<docClass>USCOURTS</docClass>
<accessId>USCOURTS-ca8-06-01807</accessId>
<courtType>Appellate</courtType>
<courtCode>ca8</courtCode>
<courtCircuit>8th</courtCircuit>
<courtSortOrder>1080</courtSortOrder>
<caseNumber>06-01807</caseNumber>
<party firstName="Sylvester" fullName="Sylvester Richards Gayekpar" lastName="Gayekpar" middleName="Richards" role="Appellee"></party>
<party fullName="United States of America" lastName="United States of America" role="Appellant"></party>
</extension>
<relatedItem type="constituent" ID="id-USCOURTS-ca8-06-01807-0" xlink:href="http://www.gpo.gov/fdsys/granule/USCOURTS-ca8-06-01807/USCOURTS-ca8-06-01807-0/mods.xml">
<titleInfo>
<title>United States v. Sylvester Gayekpar</title>
<subTitle>PER CURIAM OPINION FILED - THE COURT: ROGER L. WOLLMAN ; DAVID R. HANSEN ; STEVEN M. COLLOTON (UNPUBLISHED) [06-1595, 06-1807]</subTitle>
<partNumber>0</partNumber>
</titleInfo>
<originInfo>
<dateIssued>2007-01-04</dateIssued>
</originInfo>
<relatedItem xlink:href="http://www.gpo.gov/fdsys/pkg/pdf/USCOURTS-ca8-06-01807-0.pdf" type="otherFormat">
<identifier type="FDsys Unique ID">D09002ee180e6f2c6</identifier>
</relatedItem>
<identifier type="uri">http://www.gpo.gov/fdsys/granule/USCOURTS-ca8-06-01807/USCOURTS-ca8-06-01807-0</identifier>
<identifier type="former granule identifier">ca8-06-01807_0.pdf</identifier>
<location>
<url access="object in context" displayLabel="Content Detail">http://www.gpo.gov/fdsys/granule/USCOURTS-ca8-06-01807/USCOURTS-ca8-06-01807-0/content-detail.html</url>
<url access="raw object" displayLabel="PDF rendition">http://www.gpo.gov/fdsys/pkg/USCOURTS-ca8-06-01807/pdf/USCOURTS-ca8-06-01807-0.pdf</url>
</location>
<extension>
<searchTitle>USCOURTS 06-01807; United States v. Sylvester Gayekpar; </searchTitle>
<courtName>United States Court of Appeals for the Eighth Circuit</courtName>
<accessId>USCOURTS-ca8-06-01807-0</accessId>
<sequenceNumber>0</sequenceNumber>
<dateIssued>2007-01-04</dateIssued>
<docketText>PER CURIAM OPINION FILED - THE COURT: ROGER L. WOLLMAN ; DAVID R. HANSEN ; STEVEN M. COLLOTON (UNPUBLISHED) [06-1595, 06-1807]</docketText>
</extension>
</relatedItem>
</mods> | {
"content_hash": "64ab426dd3c3cd83f4c1a8be204890a5",
"timestamp": "",
"source": "github",
"line_count": 138,
"max_line_length": 311,
"avg_line_length": 47.028985507246375,
"alnum_prop": 0.7665639445300462,
"repo_name": "m4h7/juriscraper",
"id": "67e84ed24cfc95165eb518aad31b39aef75ea2d8",
"size": "6490",
"binary": false,
"copies": "2",
"ref": "refs/heads/master",
"path": "juriscraper/fdsys/examples/2007/ca8-06-01807.xml",
"mode": "33188",
"license": "bsd-2-clause",
"language": [
{
"name": "HTML",
"bytes": "27160373"
},
{
"name": "Makefile",
"bytes": "88"
},
{
"name": "Python",
"bytes": "623951"
}
],
"symlink_target": ""
} |
.class final Lcom/sprint/net/lte/ApnsInUse$1;
.super Ljava/lang/Object;
.source "ApnsInUse.java"
# interfaces
.implements Landroid/os/Parcelable$Creator;
# annotations
.annotation system Ldalvik/annotation/EnclosingClass;
value = Lcom/sprint/net/lte/ApnsInUse;
.end annotation
.annotation system Ldalvik/annotation/InnerClass;
accessFlags = 0x8
name = null
.end annotation
.annotation system Ldalvik/annotation/Signature;
value = {
"Ljava/lang/Object;",
"Landroid/os/Parcelable$Creator",
"<",
"Lcom/sprint/net/lte/ApnsInUse;",
">;"
}
.end annotation
# direct methods
.method constructor <init>()V
.locals 0
.prologue
.line 57
invoke-direct {p0}, Ljava/lang/Object;-><init>()V
return-void
.end method
# virtual methods
.method public createFromParcel(Landroid/os/Parcel;)Lcom/sprint/net/lte/ApnsInUse;
.locals 2
.param p1, "in" # Landroid/os/Parcel;
.prologue
.line 59
new-instance v0, Lcom/sprint/net/lte/ApnsInUse;
const/4 v1, 0x0
invoke-direct {v0, p1, v1}, Lcom/sprint/net/lte/ApnsInUse;-><init>(Landroid/os/Parcel;Lcom/sprint/net/lte/ApnsInUse$1;)V
return-object v0
.end method
.method public bridge synthetic createFromParcel(Landroid/os/Parcel;)Ljava/lang/Object;
.locals 1
.param p1, "x0" # Landroid/os/Parcel;
.prologue
.line 57
invoke-virtual {p0, p1}, Lcom/sprint/net/lte/ApnsInUse$1;->createFromParcel(Landroid/os/Parcel;)Lcom/sprint/net/lte/ApnsInUse;
move-result-object v0
return-object v0
.end method
.method public newArray(I)[Lcom/sprint/net/lte/ApnsInUse;
.locals 1
.param p1, "size" # I
.prologue
.line 63
new-array v0, p1, [Lcom/sprint/net/lte/ApnsInUse;
return-object v0
.end method
.method public bridge synthetic newArray(I)[Ljava/lang/Object;
.locals 1
.param p1, "x0" # I
.prologue
.line 57
invoke-virtual {p0, p1}, Lcom/sprint/net/lte/ApnsInUse$1;->newArray(I)[Lcom/sprint/net/lte/ApnsInUse;
move-result-object v0
return-object v0
.end method
| {
"content_hash": "2316bc4cfffd165355cbff08d5b18e0e",
"timestamp": "",
"source": "github",
"line_count": 93,
"max_line_length": 130,
"avg_line_length": 22.559139784946236,
"alnum_prop": 0.680648236415634,
"repo_name": "shumxin/FlymeOS_A5DUG",
"id": "af8de0d05c420e0a068193f01d293a14cb34f64f",
"size": "2098",
"binary": false,
"copies": "1",
"ref": "refs/heads/lollipop-5.0",
"path": "framework.jar.out/smali/com/sprint/net/lte/ApnsInUse$1.smali",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "GLSL",
"bytes": "1500"
},
{
"name": "HTML",
"bytes": "96769"
},
{
"name": "Makefile",
"bytes": "13678"
},
{
"name": "Shell",
"bytes": "103420"
},
{
"name": "Smali",
"bytes": "189389087"
}
],
"symlink_target": ""
} |
'use strict'
const postcss = require('postcss')
const LENGTH = 4000
// Get the number of selectors for a given node.
const getSelLength = node => {
if (node.type === 'rule') {
return node.selectors.length
}
if (node.type === 'atrule' && node.nodes) {
return 1 + node.nodes.reduce((memo, n) => {
return memo + getSelLength(n)
}, 0)
}
return 0
}
// 分割 css 的插件
const plugin = postcss.plugin('postcss-split-css', options => {
options = options || {}
const size = options.size || LENGTH
return (css, result) => {
const chunks = []
let chunk
// Create a new chunk that holds current result.
const nextChunk = () => {
chunk = css.clone({nodes: []})
chunk.count = 0
chunks.push(chunk)
}
// Walk the nodes. When we overflow the selector count, then start a new
// chunk. Collect the nodes into the current chunk.
css.nodes.forEach((n) => {
const selCount = getSelLength(n)
// console.log('============', n.name, selCount)
if (!chunk || chunk.count + selCount > size) {
nextChunk()
}
chunk.nodes.push(n)
chunk.count += selCount
})
// Output the results.
result.chunks = chunks.map(c => {
let ret = c.toResult({})
ret.count = c.count
return ret
})
}
})
module.exports = function (content, size) {
return postcss([plugin({ size })])
.process(content)
.then(result => {
return result.chunks.map(chunk => {
return chunk
})
})
}
| {
"content_hash": "3a4d5f43900a1cab3405b59ae2deaada",
"timestamp": "",
"source": "github",
"line_count": 65,
"max_line_length": 76,
"avg_line_length": 23.4,
"alnum_prop": 0.5746219592373438,
"repo_name": "yibn2008/css-split",
"id": "a7fc2da37c3d88fa307106e6f55106cefdd34b55",
"size": "1531",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "perf/postcss-split.js",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "CSS",
"bytes": "3685"
},
{
"name": "JavaScript",
"bytes": "8779"
}
],
"symlink_target": ""
} |
import _extends from "@babel/runtime/helpers/esm/extends";
import _objectWithoutPropertiesLoose from "@babel/runtime/helpers/esm/objectWithoutPropertiesLoose";
import * as React from 'react';
import PropTypes from 'prop-types';
import clsx from 'clsx';
import ButtonBase from '../ButtonBase';
import withStyles from '../styles/withStyles';
import AccordionContext from '../Accordion/AccordionContext';
export const styles = theme => {
const transition = {
duration: theme.transitions.duration.shortest
};
return {
/* Styles applied to the root element. */
root: {
display: 'flex',
minHeight: 8 * 6,
transition: theme.transitions.create(['min-height', 'background-color'], transition),
padding: theme.spacing(0, 2),
'&:hover:not($disabled)': {
cursor: 'pointer'
},
'&$expanded': {
minHeight: 64
},
'&$focusVisible': {
backgroundColor: theme.palette.action.focus
},
'&$disabled': {
opacity: theme.palette.action.disabledOpacity
}
},
/* Pseudo-class applied to the root element, children wrapper element and `IconButton` component if `expanded={true}`. */
expanded: {},
/* Pseudo-class applied to the ButtonBase root element if the button is keyboard focused. */
focusVisible: {},
/* Pseudo-class applied to the root element if `disabled={true}`. */
disabled: {},
/* Styles applied to the children wrapper element. */
content: {
display: 'flex',
flexGrow: 1,
transition: theme.transitions.create(['margin'], transition),
margin: '12px 0',
'&$expanded': {
margin: '20px 0'
}
},
/* Styles applied to the `expandIcon`'s wrapper element. */
expandIconWrapper: {
display: 'flex',
color: theme.palette.action.active,
transform: 'rotate(0deg)',
transition: theme.transitions.create('transform', transition),
'&$expanded': {
transform: 'rotate(180deg)'
}
}
};
};
const AccordionSummary = /*#__PURE__*/React.forwardRef(function AccordionSummary(props, ref) {
const {
children,
classes,
className,
expandIcon,
focusVisibleClassName,
onClick
} = props,
other = _objectWithoutPropertiesLoose(props, ["children", "classes", "className", "expandIcon", "focusVisibleClassName", "onClick"]);
const {
disabled = false,
expanded,
toggle
} = React.useContext(AccordionContext);
const handleChange = event => {
if (toggle) {
toggle(event);
}
if (onClick) {
onClick(event);
}
};
return /*#__PURE__*/React.createElement(ButtonBase, _extends({
focusRipple: false,
disableRipple: true,
disabled: disabled,
component: "div",
"aria-expanded": expanded,
className: clsx(classes.root, className, disabled && classes.disabled, expanded && classes.expanded),
focusVisibleClassName: clsx(classes.focusVisible, focusVisibleClassName),
onClick: handleChange,
ref: ref
}, other), /*#__PURE__*/React.createElement("div", {
className: clsx(classes.content, expanded && classes.expanded)
}, children), expandIcon && /*#__PURE__*/React.createElement("div", {
className: clsx(classes.expandIconWrapper, expanded && classes.expanded)
}, expandIcon));
});
process.env.NODE_ENV !== "production" ? AccordionSummary.propTypes = {
// ----------------------------- Warning --------------------------------
// | These PropTypes are generated from the TypeScript type definitions |
// | To update them edit the d.ts file and run "yarn proptypes" |
// ----------------------------------------------------------------------
/**
* The content of the component.
*/
children: PropTypes.node,
/**
* Override or extend the styles applied to the component.
*/
classes: PropTypes.object,
/**
* @ignore
*/
className: PropTypes.string,
/**
* The icon to display as the expand indicator.
*/
expandIcon: PropTypes.node,
/**
* This prop can help identify which element has keyboard focus.
* The class name will be applied when the element gains the focus through keyboard interaction.
* It's a polyfill for the [CSS :focus-visible selector](https://drafts.csswg.org/selectors-4/#the-focus-visible-pseudo).
* The rationale for using this feature [is explained here](https://github.com/WICG/focus-visible/blob/master/explainer.md).
* A [polyfill can be used](https://github.com/WICG/focus-visible) to apply a `focus-visible` class to other components
* if needed.
*/
focusVisibleClassName: PropTypes.string,
/**
* @ignore
*/
onClick: PropTypes.func
} : void 0;
export default withStyles(styles, {
name: 'MuiAccordionSummary'
})(AccordionSummary); | {
"content_hash": "b5ac2c79105c928413efc27f7c31316a",
"timestamp": "",
"source": "github",
"line_count": 152,
"max_line_length": 141,
"avg_line_length": 31.49342105263158,
"alnum_prop": 0.6360977647796114,
"repo_name": "cdnjs/cdnjs",
"id": "7c908b5259f0685b7cbe639936986d9ea87fbe23",
"size": "4787",
"binary": false,
"copies": "2",
"ref": "refs/heads/master",
"path": "ajax/libs/material-ui/5.0.0-alpha.23/modern/AccordionSummary/AccordionSummary.js",
"mode": "33188",
"license": "mit",
"language": [],
"symlink_target": ""
} |
namespace media {
static const int kStreamCloseDelaySeconds = 5;
// Default maximum number of output streams that can be open simultaneously
// for all platforms.
static const int kDefaultMaxOutputStreams = 16;
// Default maximum number of input streams that can be open simultaneously
// for all platforms.
static const int kDefaultMaxInputStreams = 16;
static const int kMaxInputChannels = 3;
const char AudioManagerBase::kDefaultDeviceName[] = "Default";
const char AudioManagerBase::kDefaultDeviceId[] = "default";
const char AudioManagerBase::kLoopbackInputDeviceId[] = "loopback";
struct AudioManagerBase::DispatcherParams {
DispatcherParams(const AudioParameters& input,
const AudioParameters& output,
const std::string& output_device_id)
: input_params(input),
output_params(output),
output_device_id(output_device_id) {}
~DispatcherParams() {}
const AudioParameters input_params;
const AudioParameters output_params;
const std::string output_device_id;
scoped_refptr<AudioOutputDispatcher> dispatcher;
private:
DISALLOW_COPY_AND_ASSIGN(DispatcherParams);
};
class AudioManagerBase::CompareByParams {
public:
explicit CompareByParams(const DispatcherParams* dispatcher)
: dispatcher_(dispatcher) {}
bool operator()(DispatcherParams* dispatcher_in) const {
// We will reuse the existing dispatcher when:
// 1) Unified IO is not used, input_params and output_params of the
// existing dispatcher are the same as the requested dispatcher.
// 2) Unified IO is used, input_params and output_params of the existing
// dispatcher are the same as the request dispatcher.
return (dispatcher_->input_params.Equals(dispatcher_in->input_params) &&
dispatcher_->output_params.Equals(dispatcher_in->output_params) &&
dispatcher_->output_device_id == dispatcher_in->output_device_id);
}
private:
const DispatcherParams* dispatcher_;
};
AudioManagerBase::AudioManagerBase(AudioLogFactory* audio_log_factory)
: max_num_output_streams_(kDefaultMaxOutputStreams),
max_num_input_streams_(kDefaultMaxInputStreams),
num_output_streams_(0),
num_input_streams_(0),
// TODO(dalecurtis): Switch this to an base::ObserverListThreadSafe, so we
// don't
// block the UI thread when swapping devices.
output_listeners_(
base::ObserverList<AudioDeviceListener>::NOTIFY_EXISTING_ONLY),
audio_thread_("AudioThread"),
audio_log_factory_(audio_log_factory) {
#if defined(OS_WIN)
audio_thread_.init_com_with_mta(true);
#elif defined(OS_MACOSX)
// CoreAudio calls must occur on the main thread of the process, which in our
// case is sadly the browser UI thread. Failure to execute calls on the right
// thread leads to crashes and odd behavior. See http://crbug.com/158170.
// TODO(dalecurtis): We should require the message loop to be passed in.
if (base::MessageLoopForUI::IsCurrent()) {
task_runner_ = base::ThreadTaskRunnerHandle::Get();
return;
}
#endif
CHECK(audio_thread_.Start());
task_runner_ = audio_thread_.task_runner();
}
AudioManagerBase::~AudioManagerBase() {
// The platform specific AudioManager implementation must have already
// stopped the audio thread. Otherwise, we may destroy audio streams before
// stopping the thread, resulting an unexpected behavior.
// This way we make sure activities of the audio streams are all stopped
// before we destroy them.
CHECK(!audio_thread_.IsRunning());
// All the output streams should have been deleted.
DCHECK_EQ(0, num_output_streams_);
// All the input streams should have been deleted.
DCHECK_EQ(0, num_input_streams_);
}
base::string16 AudioManagerBase::GetAudioInputDeviceModel() {
return base::string16();
}
scoped_refptr<base::SingleThreadTaskRunner> AudioManagerBase::GetTaskRunner() {
return task_runner_;
}
scoped_refptr<base::SingleThreadTaskRunner>
AudioManagerBase::GetWorkerTaskRunner() {
// Lazily start the worker thread.
if (!audio_thread_.IsRunning())
CHECK(audio_thread_.Start());
return audio_thread_.task_runner();
}
AudioOutputStream* AudioManagerBase::MakeAudioOutputStream(
const AudioParameters& params,
const std::string& device_id) {
// TODO(miu): Fix ~50 call points across several unit test modules to call
// this method on the audio thread, then uncomment the following:
// DCHECK(task_runner_->BelongsToCurrentThread());
if (!params.IsValid()) {
DLOG(ERROR) << "Audio parameters are invalid";
return NULL;
}
// Limit the number of audio streams opened. This is to prevent using
// excessive resources for a large number of audio streams. More
// importantly it prevents instability on certain systems.
// See bug: http://crbug.com/30242.
if (num_output_streams_ >= max_num_output_streams_) {
DLOG(ERROR) << "Number of opened output audio streams "
<< num_output_streams_
<< " exceed the max allowed number "
<< max_num_output_streams_;
return NULL;
}
AudioOutputStream* stream;
switch (params.format()) {
case AudioParameters::AUDIO_PCM_LINEAR:
DCHECK(device_id.empty())
<< "AUDIO_PCM_LINEAR supports only the default device.";
stream = MakeLinearOutputStream(params);
break;
case AudioParameters::AUDIO_PCM_LOW_LATENCY:
stream = MakeLowLatencyOutputStream(params, device_id);
break;
case AudioParameters::AUDIO_FAKE:
stream = FakeAudioOutputStream::MakeFakeStream(this, params);
break;
default:
stream = NULL;
break;
}
if (stream) {
++num_output_streams_;
}
return stream;
}
AudioInputStream* AudioManagerBase::MakeAudioInputStream(
const AudioParameters& params,
const std::string& device_id) {
// TODO(miu): Fix ~20 call points across several unit test modules to call
// this method on the audio thread, then uncomment the following:
// DCHECK(task_runner_->BelongsToCurrentThread());
if (!params.IsValid() || (params.channels() > kMaxInputChannels) ||
device_id.empty()) {
DLOG(ERROR) << "Audio parameters are invalid for device " << device_id;
return NULL;
}
if (num_input_streams_ >= max_num_input_streams_) {
DLOG(ERROR) << "Number of opened input audio streams "
<< num_input_streams_
<< " exceed the max allowed number " << max_num_input_streams_;
return NULL;
}
DVLOG(2) << "Creating a new AudioInputStream with buffer size = "
<< params.frames_per_buffer();
AudioInputStream* stream;
switch (params.format()) {
case AudioParameters::AUDIO_PCM_LINEAR:
stream = MakeLinearInputStream(params, device_id);
break;
case AudioParameters::AUDIO_PCM_LOW_LATENCY:
stream = MakeLowLatencyInputStream(params, device_id);
break;
case AudioParameters::AUDIO_FAKE:
stream = FakeAudioInputStream::MakeFakeStream(this, params);
break;
default:
stream = NULL;
break;
}
if (stream) {
++num_input_streams_;
}
return stream;
}
AudioOutputStream* AudioManagerBase::MakeAudioOutputStreamProxy(
const AudioParameters& params,
const std::string& device_id) {
DCHECK(task_runner_->BelongsToCurrentThread());
// If the caller supplied an empty device id to select the default device,
// we fetch the actual device id of the default device so that the lookup
// will find the correct device regardless of whether it was opened as
// "default" or via the specific id.
// NOTE: Implementations that don't yet support opening non-default output
// devices may return an empty string from GetDefaultOutputDeviceID().
std::string output_device_id = device_id.empty() ?
GetDefaultOutputDeviceID() : device_id;
// If we're not using AudioOutputResampler our output parameters are the same
// as our input parameters.
AudioParameters output_params = params;
if (params.format() == AudioParameters::AUDIO_PCM_LOW_LATENCY) {
output_params =
GetPreferredOutputStreamParameters(output_device_id, params);
// Ensure we only pass on valid output parameters.
if (!output_params.IsValid()) {
// We've received invalid audio output parameters, so switch to a mock
// output device based on the input parameters. This may happen if the OS
// provided us junk values for the hardware configuration.
LOG(ERROR) << "Invalid audio output parameters received; using fake "
<< "audio path. Channels: " << output_params.channels() << ", "
<< "Sample Rate: " << output_params.sample_rate() << ", "
<< "Bits Per Sample: " << output_params.bits_per_sample()
<< ", Frames Per Buffer: "
<< output_params.frames_per_buffer();
// Tell the AudioManager to create a fake output device.
output_params = AudioParameters(
AudioParameters::AUDIO_FAKE, params.channel_layout(),
params.sample_rate(), params.bits_per_sample(),
params.frames_per_buffer(), params.effects());
} else if (params.effects() != output_params.effects()) {
// Turn off effects that weren't requested.
output_params = AudioParameters(
output_params.format(), output_params.channel_layout(),
output_params.channels(), output_params.sample_rate(),
output_params.bits_per_sample(), output_params.frames_per_buffer(),
params.effects() & output_params.effects());
}
}
DispatcherParams* dispatcher_params =
new DispatcherParams(params, output_params, output_device_id);
AudioOutputDispatchers::iterator it =
std::find_if(output_dispatchers_.begin(), output_dispatchers_.end(),
CompareByParams(dispatcher_params));
if (it != output_dispatchers_.end()) {
delete dispatcher_params;
return new AudioOutputProxy((*it)->dispatcher.get());
}
const base::TimeDelta kCloseDelay =
base::TimeDelta::FromSeconds(kStreamCloseDelaySeconds);
scoped_refptr<AudioOutputDispatcher> dispatcher;
if (output_params.format() != AudioParameters::AUDIO_FAKE) {
dispatcher = new AudioOutputResampler(this, params, output_params,
output_device_id,
kCloseDelay);
} else {
dispatcher = new AudioOutputDispatcherImpl(this, output_params,
output_device_id,
kCloseDelay);
}
dispatcher_params->dispatcher = dispatcher;
output_dispatchers_.push_back(dispatcher_params);
return new AudioOutputProxy(dispatcher.get());
}
void AudioManagerBase::ShowAudioInputSettings() {
}
void AudioManagerBase::GetAudioInputDeviceNames(
AudioDeviceNames* device_names) {
}
void AudioManagerBase::GetAudioOutputDeviceNames(
AudioDeviceNames* device_names) {
}
void AudioManagerBase::ReleaseOutputStream(AudioOutputStream* stream) {
DCHECK(stream);
// TODO(xians) : Have a clearer destruction path for the AudioOutputStream.
// For example, pass the ownership to AudioManager so it can delete the
// streams.
--num_output_streams_;
delete stream;
}
void AudioManagerBase::ReleaseInputStream(AudioInputStream* stream) {
DCHECK(stream);
// TODO(xians) : Have a clearer destruction path for the AudioInputStream.
--num_input_streams_;
delete stream;
}
void AudioManagerBase::Shutdown() {
// Only true when we're sharing the UI message loop with the browser. The UI
// loop is no longer running at this time and browser destruction is imminent.
if (task_runner_->BelongsToCurrentThread()) {
ShutdownOnAudioThread();
} else {
task_runner_->PostTask(FROM_HERE, base::Bind(
&AudioManagerBase::ShutdownOnAudioThread, base::Unretained(this)));
}
// Stop() will wait for any posted messages to be processed first.
audio_thread_.Stop();
}
void AudioManagerBase::ShutdownOnAudioThread() {
DCHECK(task_runner_->BelongsToCurrentThread());
while (!output_dispatchers_.empty()) {
output_dispatchers_.back()->dispatcher->Shutdown();
output_dispatchers_.pop_back();
}
}
void AudioManagerBase::AddOutputDeviceChangeListener(
AudioDeviceListener* listener) {
DCHECK(task_runner_->BelongsToCurrentThread());
output_listeners_.AddObserver(listener);
}
void AudioManagerBase::RemoveOutputDeviceChangeListener(
AudioDeviceListener* listener) {
DCHECK(task_runner_->BelongsToCurrentThread());
output_listeners_.RemoveObserver(listener);
}
void AudioManagerBase::NotifyAllOutputDeviceChangeListeners() {
DCHECK(task_runner_->BelongsToCurrentThread());
DVLOG(1) << "Firing OnDeviceChange() notifications.";
FOR_EACH_OBSERVER(AudioDeviceListener, output_listeners_, OnDeviceChange());
}
AudioParameters AudioManagerBase::GetDefaultOutputStreamParameters() {
return GetPreferredOutputStreamParameters(GetDefaultOutputDeviceID(),
AudioParameters());
}
AudioParameters AudioManagerBase::GetOutputStreamParameters(
const std::string& device_id) {
return GetPreferredOutputStreamParameters(device_id,
AudioParameters());
}
AudioParameters AudioManagerBase::GetInputStreamParameters(
const std::string& device_id) {
NOTREACHED();
return AudioParameters();
}
std::string AudioManagerBase::GetAssociatedOutputDeviceID(
const std::string& input_device_id) {
return "";
}
std::string AudioManagerBase::GetDefaultOutputDeviceID() {
return "";
}
int AudioManagerBase::GetUserBufferSize() {
const base::CommandLine* cmd_line = base::CommandLine::ForCurrentProcess();
int buffer_size = 0;
std::string buffer_size_str(cmd_line->GetSwitchValueASCII(
switches::kAudioBufferSize));
if (base::StringToInt(buffer_size_str, &buffer_size) && buffer_size > 0)
return buffer_size;
return 0;
}
scoped_ptr<AudioLog> AudioManagerBase::CreateAudioLog(
AudioLogFactory::AudioComponent component) {
return audio_log_factory_->CreateAudioLog(component);
}
void AudioManagerBase::SetHasKeyboardMic() {
NOTREACHED();
}
} // namespace media
| {
"content_hash": "9237ca3d50426786e14e16f1f91d906a",
"timestamp": "",
"source": "github",
"line_count": 400,
"max_line_length": 80,
"avg_line_length": 35.47,
"alnum_prop": 0.6977022836199606,
"repo_name": "vadimtk/chrome4sdp",
"id": "ea97f1556bdafadbe31d78a1133041273ff010a8",
"size": "14945",
"binary": false,
"copies": "2",
"ref": "refs/heads/master",
"path": "media/audio/audio_manager_base.cc",
"mode": "33188",
"license": "bsd-3-clause",
"language": [],
"symlink_target": ""
} |
exports.polymorph = function(){
var len2func = [];
for(var i=0; i<arguments.length; i++)
if(typeof(arguments[i]) == "function")
len2func[arguments[i].length] = arguments[i];
return function() {
return len2func[arguments.length].apply(this, arguments);
}
}
| {
"content_hash": "96290d2d71215527c3cd46cd98a43798",
"timestamp": "",
"source": "github",
"line_count": 11,
"max_line_length": 65,
"avg_line_length": 28.454545454545453,
"alnum_prop": 0.5782747603833865,
"repo_name": "mshogin/oraclejs",
"id": "6146c463aced34fc2117d110c744209bc13b3882",
"size": "313",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "utils.js",
"mode": "33261",
"license": "mit",
"language": [
{
"name": "JavaScript",
"bytes": "8975"
}
],
"symlink_target": ""
} |
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<!--NewPage-->
<HTML>
<HEAD>
<!-- Generated by javadoc (build 1.5.0_16) on Mon Feb 16 20:04:26 EST 2009 -->
<TITLE>
All Classes
</TITLE>
<LINK REL ="stylesheet" TYPE="text/css" HREF="stylesheet.css" TITLE="Style">
</HEAD>
<BODY BGCOLOR="white">
<FONT size="+1" CLASS="FrameHeadingFont">
<B>All Classes</B></FONT>
<BR>
<TABLE BORDER="0" WIDTH="100%" SUMMARY="">
<TR>
<TD NOWRAP><FONT CLASS="FrameItemFont"><A HREF="com/mattwilliamsnyc/service/remix/Category.html" title="class in com.mattwilliamsnyc.service.remix">Category</A>
<BR>
<A HREF="com/mattwilliamsnyc/service/remix/Element.html" title="class in com.mattwilliamsnyc.service.remix">Element</A>
<BR>
<A HREF="com/mattwilliamsnyc/service/remix/Entity.html" title="class in com.mattwilliamsnyc.service.remix">Entity</A>
<BR>
<A HREF="com/mattwilliamsnyc/service/remix/ErrorDocument.html" title="class in com.mattwilliamsnyc.service.remix">ErrorDocument</A>
<BR>
<A HREF="com/mattwilliamsnyc/service/remix/Offer.html" title="class in com.mattwilliamsnyc.service.remix">Offer</A>
<BR>
<A HREF="com/mattwilliamsnyc/service/remix/Product.html" title="class in com.mattwilliamsnyc.service.remix">Product</A>
<BR>
<A HREF="com/mattwilliamsnyc/service/remix/ProductResponse.html" title="class in com.mattwilliamsnyc.service.remix">ProductResponse</A>
<BR>
<A HREF="com/mattwilliamsnyc/service/remix/ProductsResponse.html" title="class in com.mattwilliamsnyc.service.remix">ProductsResponse</A>
<BR>
<A HREF="com/mattwilliamsnyc/service/remix/RelatedItem.html" title="class in com.mattwilliamsnyc.service.remix">RelatedItem</A>
<BR>
<A HREF="com/mattwilliamsnyc/service/remix/Remix.html" title="class in com.mattwilliamsnyc.service.remix">Remix</A>
<BR>
<A HREF="com/mattwilliamsnyc/service/remix/RemixException.html" title="class in com.mattwilliamsnyc.service.remix">RemixException</A>
<BR>
<A HREF="com/mattwilliamsnyc/service/remix/util/RemixUtil.html" title="class in com.mattwilliamsnyc.service.remix.util">RemixUtil</A>
<BR>
<A HREF="com/mattwilliamsnyc/service/remix/Response.html" title="class in com.mattwilliamsnyc.service.remix">Response</A>
<BR>
<A HREF="com/mattwilliamsnyc/service/remix/Store.html" title="class in com.mattwilliamsnyc.service.remix">Store</A>
<BR>
<A HREF="com/mattwilliamsnyc/service/remix/StoreResponse.html" title="class in com.mattwilliamsnyc.service.remix">StoreResponse</A>
<BR>
<A HREF="com/mattwilliamsnyc/service/remix/StoresResponse.html" title="class in com.mattwilliamsnyc.service.remix">StoresResponse</A>
<BR>
<A HREF="com/mattwilliamsnyc/service/remix/util/XMLHandler.html" title="class in com.mattwilliamsnyc.service.remix.util">XMLHandler</A>
<BR>
</FONT></TD>
</TR>
</TABLE>
</BODY>
</HTML>
| {
"content_hash": "c4ed972516fb2dda39038bf12bdf6def",
"timestamp": "",
"source": "github",
"line_count": 62,
"max_line_length": 160,
"avg_line_length": 45.12903225806452,
"alnum_prop": 0.7634024303073624,
"repo_name": "sumit784/Best-Buy_Developer_remix-java_api",
"id": "4f715ea531297d554de4693f34b306bbf93cbada",
"size": "2798",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "doc/allclasses-noframe.html",
"mode": "33188",
"license": "bsd-3-clause",
"language": [
{
"name": "HTML",
"bytes": "4935"
},
{
"name": "Java",
"bytes": "73667"
}
],
"symlink_target": ""
} |
using System.Threading;
using Bjd;
using Xunit;
using Bjd.Initialization;
using System;
using Bjd.Threading;
using Xunit.Abstractions;
namespace Bjd.Test
{
public class ThreadBaseTest : IDisposable
{
private class MyThread : ThreadBase
{
public MyThread(Kernel kernel) : base(kernel, null)
{
}
protected override bool OnStartThread()
{
return true;
}
protected override void OnStopThread()
{
}
protected override void OnRunThread()
{
//[C#]
ThreadBaseKind = ThreadBaseKind.Running;
while (IsLife())
{
Thread.Sleep(10);
}
}
public override string GetMsg(int no)
{
return "";
}
}
TestService _service;
public ThreadBaseTest(ITestOutputHelper output)
{
_service = TestService.CreateTestService();
_service.AddOutput(output);
}
public void Dispose()
{
_service.Dispose();
}
[Fact]
public void Startする前はThreadBaseKindはBeforeとなる()
{
//setUp
using (var sut = new MyThread(_service.Kernel))
{
//var expected = ThreadBaseKind.Before;
//exercise
var actual = sut.ThreadBaseKind;
//verify
Assert.Equal(ThreadBaseKind.Before, actual);
//tearDown
}
}
[Fact]
public void StartするとThreadBaseKindはRunningとなる()
{
//setUp
using (var sut = new MyThread(_service.Kernel))
{
//var expected = ThreadBaseKind.Running;
//exercise
sut.Start();
var actual = sut.ThreadBaseKind;
//verify
Assert.Equal(ThreadBaseKind.Running, actual);
//tearDown
}
}
[Fact]
public void Startは重複しても問題ない()
{
//setUp
using (var sut = new MyThread(_service.Kernel))
{
//var expected = ThreadBaseKind.Running;
//exercise
sut.Start();
sut.Start();
sut.Start();
sut.Start(); //重複
var actual = sut.ThreadBaseKind;
//verify
Assert.Equal(ThreadBaseKind.Running, actual);
//tearDown
}
}
[Fact]
public void Stopは重複しても問題ない()
{
//setUp
using (var sut = new MyThread(_service.Kernel))
{
//var expected = ThreadBaseKind.After;
//exercise
sut.Stop(); //重複
sut.Start();
sut.Stop();
sut.Stop();
sut.Stop();
sut.Stop();
sut.Stop(); //重複
var actual = sut.ThreadBaseKind;
//verify
Assert.Equal(ThreadBaseKind.After, actual);
//tearDown
}
}
[Fact]
public void start及びstopしてisRunnigの状態を確認する_負荷テスト()
{
//setUp
using (var sut = new MyThread(_service.Kernel))
{
//exercise verify
for (var i = 0; i < 20; i++)
{
sut.Start();
Assert.Equal(ThreadBaseKind.Running, sut.ThreadBaseKind);
sut.Stop();
Assert.Equal(ThreadBaseKind.After, sut.ThreadBaseKind);
}
//tearDown
}
}
[Fact]
public void new及びstart_stop_disposeしてisRunnigの状態を確認する_負荷テスト()
{
//exercise verify
for (var i = 0; i < 20; i++)
{
var sut = new MyThread(_service.Kernel);
Assert.Equal(ThreadBaseKind.Before, sut.ThreadBaseKind);
sut.Start();
Assert.Equal(ThreadBaseKind.Running, sut.ThreadBaseKind);
sut.Stop();
Assert.Equal(ThreadBaseKind.After, sut.ThreadBaseKind);
sut.Dispose();
}
}
[Fact]
public void new及びstart_disposeしてisRunnigの状態を確認する_負荷テスト()
{
//exercise verify
for (var i = 0; i < 20; i++)
{
var sut = new MyThread(_service.Kernel);
Assert.Equal(ThreadBaseKind.Before, sut.ThreadBaseKind);
sut.Start();
Assert.Equal(ThreadBaseKind.Running, sut.ThreadBaseKind);
sut.Dispose();
Assert.Equal(ThreadBaseKind.After, sut.ThreadBaseKind);
}
}
}
} | {
"content_hash": "edab12b8b3e0e5009adbee5e3720dbfd",
"timestamp": "",
"source": "github",
"line_count": 184,
"max_line_length": 77,
"avg_line_length": 27.179347826086957,
"alnum_prop": 0.4495100979804039,
"repo_name": "darkcrash/bjd5",
"id": "3e5ee7ba0e2fe6cd20441388f72162618d541d3d",
"size": "5187",
"binary": false,
"copies": "1",
"ref": "refs/heads/CoreCLR",
"path": "Bjd.Common.Test/ThreadBaseTest.cs",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "Batchfile",
"bytes": "818"
},
{
"name": "C#",
"bytes": "2674538"
},
{
"name": "HTML",
"bytes": "792762"
},
{
"name": "PHP",
"bytes": "376"
},
{
"name": "Perl",
"bytes": "515"
},
{
"name": "Shell",
"bytes": "301"
}
],
"symlink_target": ""
} |
using System.Reflection;
using System.Runtime.CompilerServices;
using System.Runtime.InteropServices;
// General Information about an assembly is controlled through the following
// set of attributes. Change these attribute values to modify the information
// associated with an assembly.
[assembly: AssemblyTitle("11.AllPermutationsWithReps")]
[assembly: AssemblyDescription("")]
[assembly: AssemblyConfiguration("")]
[assembly: AssemblyCompany("")]
[assembly: AssemblyProduct("11.AllPermutationsWithReps")]
[assembly: AssemblyCopyright("Copyright © 2015")]
[assembly: AssemblyTrademark("")]
[assembly: AssemblyCulture("")]
// Setting ComVisible to false makes the types in this assembly not visible
// to COM components. If you need to access a type in this assembly from
// COM, set the ComVisible attribute to true on that type.
[assembly: ComVisible(false)]
// The following GUID is for the ID of the typelib if this project is exposed to COM
[assembly: Guid("f5bda3d9-7eda-462a-a89d-1e76c813f6dc")]
// Version information for an assembly consists of the following four values:
//
// Major Version
// Minor Version
// Build Number
// Revision
//
// You can specify all the values or you can default the Build and Revision Numbers
// by using the '*' as shown below:
// [assembly: AssemblyVersion("1.0.*")]
[assembly: AssemblyVersion("1.0.0.0")]
[assembly: AssemblyFileVersion("1.0.0.0")]
| {
"content_hash": "8217bd87874ee9c3b4cb8874482419d8",
"timestamp": "",
"source": "github",
"line_count": 36,
"max_line_length": 84,
"avg_line_length": 39.583333333333336,
"alnum_prop": 0.7487719298245614,
"repo_name": "zvet80/TelerikAcademyHomework",
"id": "a858eeb6cbd3af935c28a038beed5c8e173fd97f",
"size": "1428",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "12.Data Structures and Algorithms/08.Recursion/11.AllPermutationsWithReps/Properties/AssemblyInfo.cs",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "ASP",
"bytes": "334"
},
{
"name": "C#",
"bytes": "1652919"
},
{
"name": "CSS",
"bytes": "32676"
},
{
"name": "HTML",
"bytes": "106978"
},
{
"name": "JavaScript",
"bytes": "423205"
},
{
"name": "Objective-C",
"bytes": "17597"
},
{
"name": "SQLPL",
"bytes": "11561"
},
{
"name": "XSLT",
"bytes": "6789"
}
],
"symlink_target": ""
} |
$ErrorActionPreference = 'Stop';
Update-SessionEnvironment
$npmPath = Get-Command npm | ForEach-Object { $_.Path }
"Installing $env:chocolateyPackageName using nodejs..."
Start-ChocolateyProcessAsAdmin $npmPath -statements install,"-g","[email protected]"
| {
"content_hash": "c5df75c28adbc5f63ccd0998f85a9244",
"timestamp": "",
"source": "github",
"line_count": 7,
"max_line_length": 83,
"avg_line_length": 36.857142857142854,
"alnum_prop": 0.7674418604651163,
"repo_name": "chocolatey/chocolatey-coreteampackages",
"id": "5114dfacf9cceb263373c274311e280ca99257fd",
"size": "260",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "automatic/typescript/tools/chocolateyInstall.ps1",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "AutoHotkey",
"bytes": "7881"
},
{
"name": "AutoIt",
"bytes": "1828"
},
{
"name": "PowerShell",
"bytes": "785868"
}
],
"symlink_target": ""
} |
package sponsor
import "testing"
// func TestSponsorDataPath(t *testing.T) {
// t.Log("Testing sponsorDataPath function... (expected result: '" + paths.GetWebdir() + "/data/events/2018-new-york.yml')")
// testDataPath := sponsorDataPath("chef")
// if testDataPath != paths.GetWebDir()+"/data/sponsors/chef.yml" {
// t.Errorf("Expected result of '"+paths.GetWebDir()+"data/events/2018-new-york.yml' but it was %s instead.", testDataPath)
// }
// }
// func TestSponsorImagePath(t *testing.T) {
// t.Log("Testing sponsorImagePath function... (expected result: '" + webdir + "/static/img/sponsors/chef.png')")
// testImagePath := sponsorImagePath("chef")
// if testImagePath != webdir+"/static/img/sponsors/chef.png" {
// t.Errorf("Expected result of '"+webdir+"/static/img/sponsors/chef.png' but it was %s instead.", testImagePath)
// }
// }
func TestCheckSponsor(t *testing.T) {
t.Log("Testing checkSponsor function... (expected result: 'true'")
testCheckSponsor := checkSponsor("chef")
if testCheckSponsor != true {
t.Errorf("Expected result of 'true' but didn't get it")
}
}
// func TestCheckSponsorImage(t *testing.T) {
// t.Log("Testing checkSponsorImage function... (expected result: 'true'")
// s := webdir + "/static/img/sponsors/chef.png"
// testCheckSponsor := checkSponsorImage(s)
// if testCheckSponsor != true {
// t.Errorf("Expected result of 'true' but didn't get it")
// }
// }
| {
"content_hash": "208682de9f07f64d9722bc7db868f322",
"timestamp": "",
"source": "github",
"line_count": 36,
"max_line_length": 125,
"avg_line_length": 39.47222222222222,
"alnum_prop": 0.6833216045038705,
"repo_name": "mattstratton/probablyfine",
"id": "aaaeb76fae433dc9dc0e99382903fc0572886dad",
"size": "1421",
"binary": false,
"copies": "2",
"ref": "refs/heads/main",
"path": "sponsor/sponsor_test.go",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "Go",
"bytes": "72756"
},
{
"name": "Makefile",
"bytes": "1923"
}
],
"symlink_target": ""
} |
This is a port of QRoundProgressBar (https://sourceforge.net/projects/qroundprogressbar) to Python 3 PyQt5.
#### A Simple Example
Install via PyPi:
```
sudo pip install qroundprogressbar
```
Install via Git clone:
```
git clone [email protected]:ozmartian/QRoundProgressBar.git
cd QRoundProgressBar
sudo pip install .
```
Now you can use the module:
*(e.g. copy/paste this snippet of code into a demo.py file and run with 'python3 demo.py')*
```python
#!/usr/bin/env python3
# -*- coding: utf-8 -*-
import sys
import time
from PyQt5.QtCore import Qt
from PyQt5.QtGui import QBrush, QColor, QPalette
from PyQt5.QtWidgets import QApplication, qApp
from qroundprogressbar import QRoundProgressBar
app = QApplication(sys.argv)
progress = QRoundProgressBar()
progress.setBarStyle(QRoundProgressBar.BarStyle.DONUT)
# style accordingly via palette
palette = QPalette()
brush = QBrush(QColor(0, 0, 255))
brush.setStyle(Qt.SolidPattern)
palette.setBrush(QPalette.Active, QPalette.Highlight, brush)
progress.setPalette(palette)
progress.show()
# simulate delayed time that a process may take to execute
# from demonstration purposes only
for i in range(0, 100, 20):
progress.setValue(i)
qApp.processEvents()
time.sleep(3)
sys.exit(app.exec_())
```
#### A More Thorough Demo
Run TestWidget.py to see a more detailed example of the module at work.
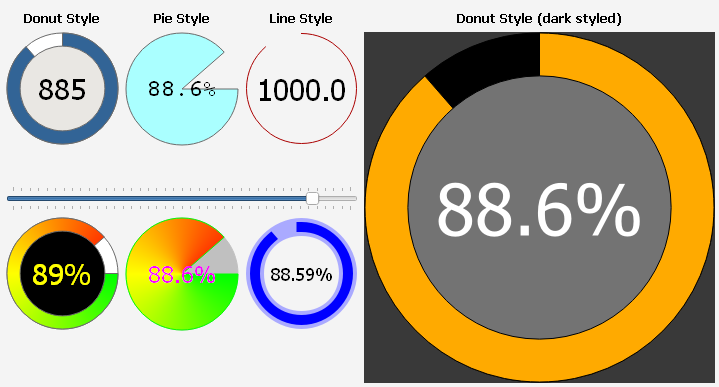
I've also included the original C++ project's documentation in the docs folder which you should easily be
able to follow and apply to PyQt5 as per usual.
| {
"content_hash": "74ddf0e666749d263ca1e3605b1803fc",
"timestamp": "",
"source": "github",
"line_count": 67,
"max_line_length": 107,
"avg_line_length": 24.01492537313433,
"alnum_prop": 0.7588564325668117,
"repo_name": "ozmartian/QRoundProgressBar",
"id": "d728e70575d5cdaf7dca4906e898b305cbb42e3f",
"size": "1609",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "README.md",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "Python",
"bytes": "39785"
}
],
"symlink_target": ""
} |
import jwt from "jsonwebtoken";
import { getSalt, getHash } from "../../../lib/promisifiedCrypto";
const config = {
hashBytes: 64,
saltBytes: 64,
iterations: 1000,
digest: "sha512"
};
export const createCredentials = async function createCredentials(password) {
try {
const salt = await getSalt(config.saltBytes);
const hash = await getHash(password, salt, config);
return {
salt,
hash: hash.toString("hex")
};
} catch (e) {
throw new Error(e);
}
};
export const validPassword = async function validPassword(
providedPassword,
{ hash, salt }
) {
const providedPasswordHash = await getHash(providedPassword, salt, config);
if (providedPasswordHash.toString("hex") !== hash) {
throw new Error("Invalid password");
}
};
export const createJwt = payload => {
const expiry = new Date();
expiry.setDate(expiry.getDate() + process.env.JWT_LIFETIME);
return jwt.sign(
{
...payload,
exp: parseInt(expiry.getTime() / 1000, 10)
},
process.env.JWT_SECRET
);
};
| {
"content_hash": "cab0d4719d0b784d99ae93018b71104f",
"timestamp": "",
"source": "github",
"line_count": 47,
"max_line_length": 77,
"avg_line_length": 22.29787234042553,
"alnum_prop": 0.6526717557251909,
"repo_name": "lexnapoles/bayuk",
"id": "94e315925bfd53ec40ecdf0fad17c1c42c99dff2",
"size": "1048",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "server/api/services/authentication.js",
"mode": "33188",
"license": "mit",
"language": [],
"symlink_target": ""
} |
Version
-------
This key is **required**.
Version of the image.
.. code:: yaml
version: "1.4"
| {
"content_hash": "b03c3da42666a6388842de39b299ac43",
"timestamp": "",
"source": "github",
"line_count": 11,
"max_line_length": 25,
"avg_line_length": 9.363636363636363,
"alnum_prop": 0.5631067961165048,
"repo_name": "jboss-container-images/concreate",
"id": "a1cbcee9c024e3c6c70623cf8f5591db38c5ccdc",
"size": "103",
"binary": false,
"copies": "1",
"ref": "refs/heads/develop",
"path": "docs/descriptor/version.rst",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "C",
"bytes": "48"
},
{
"name": "HTML",
"bytes": "3182"
},
{
"name": "Makefile",
"bytes": "747"
},
{
"name": "Python",
"bytes": "90236"
}
],
"symlink_target": ""
} |
"""
docstring goes here.
:copyright: Copyright 2014 by the Elephant team, see AUTHORS.txt.
:license: Modified BSD, see LICENSE.txt for details.
"""
import unittest
import neo
import numpy as np
from numpy.testing.utils import assert_array_almost_equal
import quantities as pq
import elephant.statistics as es
class isi_TestCase(unittest.TestCase):
def setUp(self):
self.test_array_2d = np.array([[0.3, 0.56, 0.87, 1.23],
[0.02, 0.71, 1.82, 8.46],
[0.03, 0.14, 0.15, 0.92]])
self.targ_array_2d_0 = np.array([[-0.28, 0.15, 0.95, 7.23],
[0.01, -0.57, -1.67, -7.54]])
self.targ_array_2d_1 = np.array([[0.26, 0.31, 0.36],
[0.69, 1.11, 6.64],
[0.11, 0.01, 0.77]])
self.targ_array_2d_default = self.targ_array_2d_1
self.test_array_1d = self.test_array_2d[0, :]
self.targ_array_1d = self.targ_array_2d_1[0, :]
def test_isi_with_spiketrain(self):
st = neo.SpikeTrain(self.test_array_1d, units='ms', t_stop=10.0)
target = pq.Quantity(self.targ_array_1d, 'ms')
res = es.isi(st)
assert_array_almost_equal(res, target, decimal=9)
def test_isi_with_quantities_1d(self):
st = pq.Quantity(self.test_array_1d, units='ms')
target = pq.Quantity(self.targ_array_1d, 'ms')
res = es.isi(st)
assert_array_almost_equal(res, target, decimal=9)
def test_isi_with_plain_array_1d(self):
st = self.test_array_1d
target = self.targ_array_1d
res = es.isi(st)
assert not isinstance(res, pq.Quantity)
assert_array_almost_equal(res, target, decimal=9)
def test_isi_with_plain_array_2d_default(self):
st = self.test_array_2d
target = self.targ_array_2d_default
res = es.isi(st)
assert not isinstance(res, pq.Quantity)
assert_array_almost_equal(res, target, decimal=9)
def test_isi_with_plain_array_2d_0(self):
st = self.test_array_2d
target = self.targ_array_2d_0
res = es.isi(st, axis=0)
assert not isinstance(res, pq.Quantity)
assert_array_almost_equal(res, target, decimal=9)
def test_isi_with_plain_array_2d_1(self):
st = self.test_array_2d
target = self.targ_array_2d_1
res = es.isi(st, axis=1)
assert not isinstance(res, pq.Quantity)
assert_array_almost_equal(res, target, decimal=9)
class isi_cv_TestCase(unittest.TestCase):
def setUp(self):
self.test_array_regular = np.arange(1, 6)
def test_cv_isi_regular_spiketrain_is_zero(self):
st = neo.SpikeTrain(self.test_array_regular, units='ms', t_stop=10.0)
targ = 0.0
res = es.cv(es.isi(st))
self.assertEqual(res, targ)
def test_cv_isi_regular_array_is_zero(self):
st = self.test_array_regular
targ = 0.0
res = es.cv(es.isi(st))
self.assertEqual(res, targ)
class mean_firing_rate_TestCase(unittest.TestCase):
def setUp(self):
self.test_array_3d = np.ones([5, 7, 13])
self.test_array_2d = np.array([[0.3, 0.56, 0.87, 1.23],
[0.02, 0.71, 1.82, 8.46],
[0.03, 0.14, 0.15, 0.92]])
self.targ_array_2d_0 = np.array([3, 3, 3, 3])
self.targ_array_2d_1 = np.array([4, 4, 4])
self.targ_array_2d_None = 12
self.targ_array_2d_default = self.targ_array_2d_None
self.max_array_2d_0 = np.array([0.3, 0.71, 1.82, 8.46])
self.max_array_2d_1 = np.array([1.23, 8.46, 0.92])
self.max_array_2d_None = 8.46
self.max_array_2d_default = self.max_array_2d_None
self.test_array_1d = self.test_array_2d[0, :]
self.targ_array_1d = self.targ_array_2d_1[0]
self.max_array_1d = self.max_array_2d_1[0]
def test_mean_firing_rate_with_spiketrain(self):
st = neo.SpikeTrain(self.test_array_1d, units='ms', t_stop=10.0)
target = pq.Quantity(self.targ_array_1d/10., '1/ms')
res = es.mean_firing_rate(st)
assert_array_almost_equal(res, target, decimal=9)
def test_mean_firing_rate_with_spiketrain_set_ends(self):
st = neo.SpikeTrain(self.test_array_1d, units='ms', t_stop=10.0)
target = pq.Quantity(2/0.5, '1/ms')
res = es.mean_firing_rate(st, t_start=0.4, t_stop=0.9)
assert_array_almost_equal(res, target, decimal=9)
def test_mean_firing_rate_with_quantities_1d(self):
st = pq.Quantity(self.test_array_1d, units='ms')
target = pq.Quantity(self.targ_array_1d/self.max_array_1d, '1/ms')
res = es.mean_firing_rate(st)
assert_array_almost_equal(res, target, decimal=9)
def test_mean_firing_rate_with_quantities_1d_set_ends(self):
st = pq.Quantity(self.test_array_1d, units='ms')
target = pq.Quantity(2/0.6, '1/ms')
res = es.mean_firing_rate(st, t_start=400*pq.us, t_stop=1.)
assert_array_almost_equal(res, target, decimal=9)
def test_mean_firing_rate_with_plain_array_1d(self):
st = self.test_array_1d
target = self.targ_array_1d/self.max_array_1d
res = es.mean_firing_rate(st)
assert not isinstance(res, pq.Quantity)
assert_array_almost_equal(res, target, decimal=9)
def test_mean_firing_rate_with_plain_array_1d_set_ends(self):
st = self.test_array_1d
target = self.targ_array_1d/(1.23-0.3)
res = es.mean_firing_rate(st, t_start=0.3, t_stop=1.23)
assert not isinstance(res, pq.Quantity)
assert_array_almost_equal(res, target, decimal=9)
def test_mean_firing_rate_with_plain_array_2d_default(self):
st = self.test_array_2d
target = self.targ_array_2d_default/self.max_array_2d_default
res = es.mean_firing_rate(st)
assert not isinstance(res, pq.Quantity)
assert_array_almost_equal(res, target, decimal=9)
def test_mean_firing_rate_with_plain_array_2d_0(self):
st = self.test_array_2d
target = self.targ_array_2d_0/self.max_array_2d_0
res = es.mean_firing_rate(st, axis=0)
assert not isinstance(res, pq.Quantity)
assert_array_almost_equal(res, target, decimal=9)
def test_mean_firing_rate_with_plain_array_2d_1(self):
st = self.test_array_2d
target = self.targ_array_2d_1/self.max_array_2d_1
res = es.mean_firing_rate(st, axis=1)
assert not isinstance(res, pq.Quantity)
assert_array_almost_equal(res, target, decimal=9)
def test_mean_firing_rate_with_plain_array_3d_None(self):
st = self.test_array_3d
target = np.sum(self.test_array_3d, None)/5.
res = es.mean_firing_rate(st, axis=None, t_stop=5.)
assert not isinstance(res, pq.Quantity)
assert_array_almost_equal(res, target, decimal=9)
def test_mean_firing_rate_with_plain_array_3d_0(self):
st = self.test_array_3d
target = np.sum(self.test_array_3d, 0)/5.
res = es.mean_firing_rate(st, axis=0, t_stop=5.)
assert not isinstance(res, pq.Quantity)
assert_array_almost_equal(res, target, decimal=9)
def test_mean_firing_rate_with_plain_array_3d_1(self):
st = self.test_array_3d
target = np.sum(self.test_array_3d, 1)/5.
res = es.mean_firing_rate(st, axis=1, t_stop=5.)
assert not isinstance(res, pq.Quantity)
assert_array_almost_equal(res, target, decimal=9)
def test_mean_firing_rate_with_plain_array_3d_2(self):
st = self.test_array_3d
target = np.sum(self.test_array_3d, 2)/5.
res = es.mean_firing_rate(st, axis=2, t_stop=5.)
assert not isinstance(res, pq.Quantity)
assert_array_almost_equal(res, target, decimal=9)
def test_mean_firing_rate_with_plain_array_2d_1_set_ends(self):
st = self.test_array_2d
target = np.array([4, 1, 3])/(1.23-0.14)
res = es.mean_firing_rate(st, axis=1, t_start=0.14, t_stop=1.23)
assert not isinstance(res, pq.Quantity)
assert_array_almost_equal(res, target, decimal=9)
def test_mean_firing_rate_with_plain_array_2d_None(self):
st = self.test_array_2d
target = self.targ_array_2d_None/self.max_array_2d_None
res = es.mean_firing_rate(st, axis=None)
assert not isinstance(res, pq.Quantity)
assert_array_almost_equal(res, target, decimal=9)
def test_mean_firing_rate_with_plain_array_and_units_start_stop_typeerror(self):
st = self.test_array_2d
self.assertRaises(TypeError, es.mean_firing_rate, st,
t_start=pq.Quantity(0, 'ms'))
self.assertRaises(TypeError, es.mean_firing_rate, st,
t_stop=pq.Quantity(10, 'ms'))
self.assertRaises(TypeError, es.mean_firing_rate, st,
t_start=pq.Quantity(0, 'ms'),
t_stop=pq.Quantity(10, 'ms'))
self.assertRaises(TypeError, es.mean_firing_rate, st,
t_start=pq.Quantity(0, 'ms'),
t_stop=10.)
self.assertRaises(TypeError, es.mean_firing_rate, st,
t_start=0.,
t_stop=pq.Quantity(10, 'ms'))
class FanoFactorTestCase(unittest.TestCase):
def setUp(self):
np.random.seed(100)
num_st = 300
self.test_spiketrains = []
self.test_array = []
self.test_quantity = []
self.test_list = []
self.sp_counts = np.zeros(num_st)
for i in range(num_st):
r = np.random.rand(np.random.randint(20) + 1)
st = neo.core.SpikeTrain(r * pq.ms,
t_start=0.0 * pq.ms,
t_stop=20.0 * pq.ms)
self.test_spiketrains.append(st)
self.test_array.append(r)
self.test_quantity.append(r * pq.ms)
self.test_list.append(list(r))
# for cross-validation
self.sp_counts[i] = len(st)
def test_fanofactor_spiketrains(self):
# Test with list of spiketrains
self.assertEqual(
np.var(self.sp_counts) / np.mean(self.sp_counts),
es.fanofactor(self.test_spiketrains))
# One spiketrain in list
st = self.test_spiketrains[0]
self.assertEqual(es.fanofactor([st]), 0.0)
def test_fanofactor_empty(self):
# Test with empty list
self.assertTrue(np.isnan(es.fanofactor([])))
self.assertTrue(np.isnan(es.fanofactor([[]])))
# Test with empty quantity
self.assertTrue(np.isnan(es.fanofactor([] * pq.ms)))
# Empty spiketrain
st = neo.core.SpikeTrain([] * pq.ms, t_start=0 * pq.ms,
t_stop=1.5 * pq.ms)
self.assertTrue(np.isnan(es.fanofactor(st)))
def test_fanofactor_spiketrains_same(self):
# Test with same spiketrains in list
sts = [self.test_spiketrains[0]] * 3
self.assertEqual(es.fanofactor(sts), 0.0)
def test_fanofactor_array(self):
self.assertEqual(es.fanofactor(self.test_array),
np.var(self.sp_counts) / np.mean(self.sp_counts))
def test_fanofactor_array_same(self):
lst = [self.test_array[0]] * 3
self.assertEqual(es.fanofactor(lst), 0.0)
def test_fanofactor_quantity(self):
self.assertEqual(es.fanofactor(self.test_quantity),
np.var(self.sp_counts) / np.mean(self.sp_counts))
def test_fanofactor_quantity_same(self):
lst = [self.test_quantity[0]] * 3
self.assertEqual(es.fanofactor(lst), 0.0)
def test_fanofactor_list(self):
self.assertEqual(es.fanofactor(self.test_list),
np.var(self.sp_counts) / np.mean(self.sp_counts))
def test_fanofactor_list_same(self):
lst = [self.test_list[0]] * 3
self.assertEqual(es.fanofactor(lst), 0.0)
if __name__ == '__main__':
unittest.main()
| {
"content_hash": "99e5c266cf693d9e458f588f49b42cb5",
"timestamp": "",
"source": "github",
"line_count": 304,
"max_line_length": 84,
"avg_line_length": 39.91776315789474,
"alnum_prop": 0.5855789039967038,
"repo_name": "SPP1665DataAnalysisCourse/elephant",
"id": "f23c2073900a6ec438f8e457cd4cb4f40545d46a",
"size": "12159",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "elephant/test/test_statistics.py",
"mode": "33188",
"license": "bsd-3-clause",
"language": [
{
"name": "Makefile",
"bytes": "5572"
},
{
"name": "Python",
"bytes": "522171"
},
{
"name": "Shell",
"bytes": "8849"
}
],
"symlink_target": ""
} |
//%LICENSE////////////////////////////////////////////////////////////////
//
// Licensed to The Open Group (TOG) under one or more contributor license
// agreements. Refer to the OpenPegasusNOTICE.txt file distributed with
// this work for additional information regarding copyright ownership.
// Each contributor licenses this file to you under the OpenPegasus Open
// Source License; you may not use this file except in compliance with the
// License.
//
// Permission is hereby granted, free of charge, to any person obtaining a
// copy of this software and associated documentation files (the "Software"),
// to deal in the Software without restriction, including without limitation
// the rights to use, copy, modify, merge, publish, distribute, sublicense,
// and/or sell copies of the Software, and to permit persons to whom the
// Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included
// in all copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS
// OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
// MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
// IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY
// CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
// TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
// SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
//
//////////////////////////////////////////////////////////////////////////
//
//%/////////////////////////////////////////////////////////////////////////
#include "UNIX_TextRedirectionSAP.h"
#if defined(PEGASUS_OS_HPUX)
# include "UNIX_TextRedirectionSAP_HPUX.hxx"
# include "UNIX_TextRedirectionSAP_HPUX.hpp"
#elif defined(PEGASUS_OS_LINUX)
# include "UNIX_TextRedirectionSAP_LINUX.hxx"
# include "UNIX_TextRedirectionSAP_LINUX.hpp"
#elif defined(PEGASUS_OS_DARWIN)
# include "UNIX_TextRedirectionSAP_DARWIN.hxx"
# include "UNIX_TextRedirectionSAP_DARWIN.hpp"
#elif defined(PEGASUS_OS_AIX)
# include "UNIX_TextRedirectionSAP_AIX.hxx"
# include "UNIX_TextRedirectionSAP_AIX.hpp"
#elif defined(PEGASUS_OS_FREEBSD)
# include "UNIX_TextRedirectionSAP_FREEBSD.hxx"
# include "UNIX_TextRedirectionSAP_FREEBSD.hpp"
#elif defined(PEGASUS_OS_SOLARIS)
# include "UNIX_TextRedirectionSAP_SOLARIS.hxx"
# include "UNIX_TextRedirectionSAP_SOLARIS.hpp"
#elif defined(PEGASUS_OS_ZOS)
# include "UNIX_TextRedirectionSAP_ZOS.hxx"
# include "UNIX_TextRedirectionSAP_ZOS.hpp"
#elif defined(PEGASUS_OS_VMS)
# include "UNIX_TextRedirectionSAP_VMS.hxx"
# include "UNIX_TextRedirectionSAP_VMS.hpp"
#elif defined(PEGASUS_OS_TRU64)
# include "UNIX_TextRedirectionSAP_TRU64.hxx"
# include "UNIX_TextRedirectionSAP_TRU64.hpp"
#else
# include "UNIX_TextRedirectionSAP_STUB.hxx"
# include "UNIX_TextRedirectionSAP_STUB.hpp"
#endif
Boolean UNIX_TextRedirectionSAP::validateKey(CIMKeyBinding &kb) const
{
/* Keys */
//SystemCreationClassName
//SystemName
//CreationClassName
//Name
CIMName name = kb.getName();
if (name.equal(PROPERTY_SYSTEM_CREATION_CLASS_NAME) ||
name.equal(PROPERTY_SYSTEM_NAME) ||
name.equal(PROPERTY_CREATION_CLASS_NAME) ||
name.equal(PROPERTY_NAME)
)
return true;
return false;
}
void UNIX_TextRedirectionSAP::setScope(CIMName scope)
{
currentScope = scope;
}
| {
"content_hash": "6c185fcd4bf0c9a05186270ce33bbbb4",
"timestamp": "",
"source": "github",
"line_count": 91,
"max_line_length": 77,
"avg_line_length": 37.582417582417584,
"alnum_prop": 0.7228070175438597,
"repo_name": "brunolauze/openpegasus-providers-old",
"id": "4f026bd96525c7c35f211a6dc96bf7bf13ed458b",
"size": "3420",
"binary": false,
"copies": "2",
"ref": "refs/heads/master",
"path": "src/Providers/UNIXProviders/TextRedirectionSAP/UNIX_TextRedirectionSAP.cpp",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "C",
"bytes": "2631164"
},
{
"name": "C++",
"bytes": "120884401"
},
{
"name": "Objective-C",
"bytes": "64"
},
{
"name": "Shell",
"bytes": "17094"
}
],
"symlink_target": ""
} |
<link rel="import" href="../../bower_components/polymer/polymer.html">
<dom-module id="create-meetup">
<style>
#slider {
width: 100%;
--paper-slider-disabled-active-color: --google-blue-700;
}
.page-title {
margin-bottom: 0;
}
paper-button {
width: 100%;
background: #2E3AA1;
color: black;
}
paper-button:hover {
background: #3372DF;
}
paper-button[disabled],
paper-button[toggles][active] {
background: #fafafa;
color: gray;
}
</style>
<template>
<paper-progress id="slider"></paper-progress>
<h2 class="page-title">New Meetup</h2>
<paper-input label="What are we calling this event?" value="{{params.eventName::input}}"></paper-input>
<paper-input label="What type of event is this?" value="{{params.eventType::input}}"></paper-input>
<paper-input label="Who will be hosting this event?" value="{{params.eventHost::input}}"></paper-input>
<paper-input label="Who will be attending this event?"></paper-input>
<h3 class="page-title">Details</h3>
<paper-input label="Where is the meetup located?" value="{{params.eventLocation::input}}"></paper-input>
<paper-input label="Event start date and time" value="{{params.eventStartTime::input}}" style="width: 30%; display: inline-block;"></paper-input>
<paper-input label="Event end date and time" value="{{params.eventEndTime::input}}" style="width: 30%; display: inline-block;"></paper-input>
<paper-button id="create-meetup-btn" raised on-tap="createMeetupHandler" disabled="{{computeCreateEventDisabled(params.name)}}">Create</paper-button>
</template>
<script>
(function () {
'use strict'
Polymer({
is: 'create-meetup',
properties: {
params: {
type: Object,
value: {
eventName: '',
eventType: '',
eventHost: '',
eventLocation: '',
eventStartTime: '',
eventEndTime: ''
}
},
validation: {
type: Object,
value: {
name: {
type: Boolean
},
email: {
type: Boolean
}
}
}
},
validateForm: function (params) {
if(!params) return;
// name
// host
// time
// endtime
// location ( request then confirm through google)
// event type (array search)
//
//
//
// d
//Check if form is valid then adjust progress bar based on validaty then
//open notification box showing value errors
//Adjust progress bar based on the form validation status
//If form valid return true and make create event button clickable
},
createMeetupHandler: function (e) {
//Button was successfully clicked.
//revalidate form, if form is valid send data to firebase
//parse firebase response add event data to database
},
computeCreateEventDisabled: function (params) {
//call validate form
//if form is valid make button clickable
}
})
})()
</script>
</dom-module>
| {
"content_hash": "5e8adf97572268b697318a1af31579b3",
"timestamp": "",
"source": "github",
"line_count": 108,
"max_line_length": 153,
"avg_line_length": 29.75,
"alnum_prop": 0.5742296918767507,
"repo_name": "mikelseverson/Meetup_Planner",
"id": "2ce1f2b87366c97a10be011da99ce6e45abbf3d9",
"size": "3213",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "app/elements/create-meetup/create-meetup.html",
"mode": "33188",
"license": "bsd-3-clause",
"language": [
{
"name": "CSS",
"bytes": "120"
},
{
"name": "HTML",
"bytes": "32079"
},
{
"name": "JavaScript",
"bytes": "13696"
},
{
"name": "Shell",
"bytes": "2316"
}
],
"symlink_target": ""
} |
package org.apache.geode.internal.protocol.exception;
import org.apache.geode.annotations.Experimental;
/**
* Indicates that a message didn't properly follow its protocol specification.
*/
@Experimental
public class InvalidProtocolMessageException extends Exception {
public InvalidProtocolMessageException(String message) {
super(message);
}
public InvalidProtocolMessageException(String message, Throwable cause) {
super(message, cause);
}
}
| {
"content_hash": "17b40e42ebc0d259c9946a4cd9b2e4bd",
"timestamp": "",
"source": "github",
"line_count": 18,
"max_line_length": 78,
"avg_line_length": 25.88888888888889,
"alnum_prop": 0.7854077253218884,
"repo_name": "pivotal-amurmann/geode",
"id": "49786f8c80435ec424893998aff952b63ee4d4b4",
"size": "1255",
"binary": false,
"copies": "2",
"ref": "refs/heads/develop",
"path": "geode-protobuf/src/main/java/org/apache/geode/internal/protocol/exception/InvalidProtocolMessageException.java",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "CSS",
"bytes": "106707"
},
{
"name": "Groovy",
"bytes": "2928"
},
{
"name": "HTML",
"bytes": "4012081"
},
{
"name": "Java",
"bytes": "27165660"
},
{
"name": "JavaScript",
"bytes": "1781013"
},
{
"name": "Protocol Buffer",
"bytes": "9658"
},
{
"name": "Ruby",
"bytes": "6677"
},
{
"name": "Shell",
"bytes": "21474"
}
],
"symlink_target": ""
} |
package com.kotcrab.vis.editor.event;
/**
* Posted when scene should reloed various types of resources
* @author Kotcrab
*/
public class ResourceReloadedEvent {
public static final int RESOURCE_TEXTURES = 0x0001;
public static final int RESOURCE_TEXTURE_ATLASES = 0x0002;
public static final int RESOURCE_PARTICLES = 0x0004;
public static final int RESOURCE_BMP_FONTS = 0x0008;
public static final int RESOURCE_TTF_FONTS = 0x0016;
public static final int RESOURCE_SHADERS = 0x0032;
public final int resourceType;
public ResourceReloadedEvent (int resourceType) {
this.resourceType = resourceType;
}
}
| {
"content_hash": "36a7a3aeb2894d06759f4c5b663d787d",
"timestamp": "",
"source": "github",
"line_count": 22,
"max_line_length": 61,
"avg_line_length": 28.181818181818183,
"alnum_prop": 0.7741935483870968,
"repo_name": "billy1380/VisEditor",
"id": "bb8d7e1cdae0c10afeedd68fb26de633a297a0c3",
"size": "1221",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "Editor/src/com/kotcrab/vis/editor/event/ResourceReloadedEvent.java",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "Java",
"bytes": "672251"
}
],
"symlink_target": ""
} |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Codebird Demo</title>
<script src="../bower_components/angular/angular.min.js"></script>
<script src="../bower_components/apiNG/dist/aping.min.js"></script>
<script src="aping-config.js"></script>
<script src="app.js"></script>
<script src="../dist/aping-plugin-codebird.js"></script>
</head>
<body ng-app="app">
<h1>{'search':'münchen', 'result_type':'recent', 'language':'de'}</h1>
<aping
template-url="template.html"
model="image"
aping-codebird="[{'search':'münchen', 'result_type':'recent', 'language':'de', 'items':40}]">
</aping>
<hr>
<h1>{'search':'münchen', 'result_type':'recent', 'lat':'48.1374300', 'lng':'11.5754900', 'distance':5}</h1>
<aping template-url="template.html" model="social" aping-codebird="[{'search':'münchen', 'result_type':'recent', 'lat':'48.1374300', 'lng':'11.5754900', 'distance':5}]"></aping>
<hr>
<h1>{'user':'passy', 'showAvatar':false, 'exclude_replies':true, 'include_rts':false}</h1>
<aping template-url="template.html" model="social" aping-codebird="[{'user':'passy', 'showAvatar':false, 'exclude_replies':false, 'include_rts':false, 'items':30}]"></aping>
</body>
</html> | {
"content_hash": "e9804d9690b04a31dc59cafe5220f57c",
"timestamp": "",
"source": "github",
"line_count": 28,
"max_line_length": 181,
"avg_line_length": 45.892857142857146,
"alnum_prop": 0.6140077821011674,
"repo_name": "JohnnyTheTank/apiNG-plugin-codebird",
"id": "9f43a490fb2bb2eb9e982be0470ef1fe8ccf54a6",
"size": "1289",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "demo/index.html",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "JavaScript",
"bytes": "15653"
}
],
"symlink_target": ""
} |
"""Spectrogram augmentation for model regularization."""
from typing import Any, Dict
import tensorflow_model_optimization as tfmot
from kws_streaming.layers.compat import tf
from tensorflow.python.keras.utils import control_flow_util # pylint: disable=g-direct-tensorflow-import
from tensorflow.python.ops import array_ops # pylint: disable=g-direct-tensorflow-import
def spectrogram_masking(spectrogram, dim=1, masks_number=2, mask_max_size=5):
"""Spectrogram masking on frequency or time dimension.
Args:
spectrogram: Input spectrum [batch, time, frequency]
dim: dimension on which masking will be applied: 1 - time; 2 - frequency
masks_number: number of masks
mask_max_size: mask max size
Returns:
masked spectrogram
"""
if dim not in (1, 2):
raise ValueError('Wrong dim value: %d' % dim)
input_shape = spectrogram.shape
time_size, frequency_size = input_shape[1:3]
dim_size = input_shape[dim] # size of dimension on which mask is applied
stripe_shape = [1, time_size, frequency_size]
for _ in range(masks_number):
mask_end = tf.random.uniform([], 0, mask_max_size, tf.int32)
mask_start = tf.random.uniform([], 0, dim_size - mask_end, tf.int32)
# initialize stripes with stripe_shape
stripe_ones_left = list(stripe_shape)
stripe_zeros_center = list(stripe_shape)
stripe_ones_right = list(stripe_shape)
# update stripes dim
stripe_ones_left[dim] = dim_size - mask_start - mask_end
stripe_zeros_center[dim] = mask_end
stripe_ones_right[dim] = mask_start
# generate mask
mask = tf.concat((
tf.ones(stripe_ones_left, spectrogram.dtype),
tf.zeros(stripe_zeros_center, spectrogram.dtype),
tf.ones(stripe_ones_right, spectrogram.dtype),
), dim)
spectrogram = spectrogram * mask
return spectrogram
class SpecAugment(tf.keras.layers.Layer):
"""Spectrogram augmentation.
It is based on paper: SpecAugment: A Simple Data Augmentation Method
for Automatic Speech Recognition https://arxiv.org/pdf/1904.08779.pdf
"""
def __init__(self,
time_masks_number=2,
time_mask_max_size=10,
frequency_masks_number=2,
frequency_mask_max_size=5,
**kwargs):
super(SpecAugment, self).__init__(**kwargs)
self.time_mask_max_size = time_mask_max_size
self.time_masks_number = time_masks_number
self.frequency_mask_max_size = frequency_mask_max_size
self.frequency_masks_number = frequency_masks_number
def call(self, inputs, training=None):
if training is None:
training = tf.keras.backend.learning_phase()
def masked_inputs():
# in time dim
net = spectrogram_masking(inputs, 1, self.time_masks_number,
self.time_mask_max_size)
# in frequency dim
net = spectrogram_masking(net, 2, self.frequency_masks_number,
self.frequency_mask_max_size)
return net
outputs = control_flow_util.smart_cond(training, masked_inputs,
lambda: array_ops.identity(inputs))
return outputs
def get_config(self):
config = {
'frequency_masks_number': self.frequency_masks_number,
'frequency_mask_max_size': self.frequency_mask_max_size,
'time_masks_number': self.time_masks_number,
'time_mask_max_size': self.time_mask_max_size,
}
base_config = super(SpecAugment, self).get_config()
return dict(list(base_config.items()) + list(config.items()))
# Quantization aware training support for custom operations.
def quantizable_spectrogram_augment(is_quantize = False,
**kwargs):
"""Functional API with quantization annotations."""
if is_quantize:
return tfmot.quantization.keras.quantize_annotate_layer(
SpecAugment(**kwargs), DoNotQuantizeConfig())
else:
return SpecAugment(**kwargs)
class DoNotQuantizeConfig(tfmot.quantization.keras.QuantizeConfig):
"""QuantizeConfig which does not quantize any part of the layer."""
def get_weights_and_quantizers(
self, layer
):
return []
def get_activations_and_quantizers(
self, layer
):
return []
def set_quantize_weights(self, layer,
quantize_weights):
return []
def set_quantize_activations(
self, layer, quantize_activations
):
return []
def get_output_quantizers(
self, layer
):
return []
def get_config(self):
return {}
def quantization_scopes():
"""Quantization scope for known custom operations."""
return {
'SpecAugment': SpecAugment,
'DoNotQuantizeConfig': DoNotQuantizeConfig,
}
| {
"content_hash": "2a85b82eb8c70b3d74a56abb21ef8cc2",
"timestamp": "",
"source": "github",
"line_count": 148,
"max_line_length": 105,
"avg_line_length": 31.89864864864865,
"alnum_prop": 0.6613005719127304,
"repo_name": "google-research/google-research",
"id": "6db8b6b8c890c18ca2ef7bd55064f0e17d932083",
"size": "5329",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "kws_streaming/layers/spectrogram_augment.py",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "C",
"bytes": "9817"
},
{
"name": "C++",
"bytes": "4166670"
},
{
"name": "CMake",
"bytes": "6412"
},
{
"name": "CSS",
"bytes": "27092"
},
{
"name": "Cuda",
"bytes": "1431"
},
{
"name": "Dockerfile",
"bytes": "7145"
},
{
"name": "Gnuplot",
"bytes": "11125"
},
{
"name": "HTML",
"bytes": "77599"
},
{
"name": "ImageJ Macro",
"bytes": "50488"
},
{
"name": "Java",
"bytes": "487585"
},
{
"name": "JavaScript",
"bytes": "896512"
},
{
"name": "Julia",
"bytes": "67986"
},
{
"name": "Jupyter Notebook",
"bytes": "71290299"
},
{
"name": "Lua",
"bytes": "29905"
},
{
"name": "MATLAB",
"bytes": "103813"
},
{
"name": "Makefile",
"bytes": "5636"
},
{
"name": "NASL",
"bytes": "63883"
},
{
"name": "Perl",
"bytes": "8590"
},
{
"name": "Python",
"bytes": "53790200"
},
{
"name": "R",
"bytes": "101058"
},
{
"name": "Roff",
"bytes": "1208"
},
{
"name": "Rust",
"bytes": "2389"
},
{
"name": "Shell",
"bytes": "730444"
},
{
"name": "Smarty",
"bytes": "5966"
},
{
"name": "Starlark",
"bytes": "245038"
}
],
"symlink_target": ""
} |
require 'rubygems'
require 'packetfu'
# A factory for generating packet objects on demand
module BNAT
class PacketFactory
# @param [String] a string of the interface name
def initialize(interface)
@interface = interface
end
#Get a Generic TCP Packet
def get_tcp_packet
tcp_packet = PacketFu::TCPPacket.new(
:config=> PacketFu::Utils.whoami?(:iface => "#{@interface}"),
:timeout=> 0.1,
:flavor=>"Windows"
)
return tcp_packet
end
# Get a TCP SYN Probe Packet
# @param opts [Integer] :port the destination port to target
# @param opts [String] :ip_daddr the destination port to target
def get_syn_probe(opts = {})
tcp_syn_probe = get_tcp_packet()
tcp_syn_probe.tcp_flags.syn=1
tcp_syn_probe.tcp_win=14600
tcp_syn_probe.tcp_options="MSS:1460,SACKOK,TS:3853;0,NOP,WS:5"
tcp_syn_probe.tcp_src = rand(64511)+1024
tcp_syn_probe.tcp_seq = rand(2**32-10**9)+10**9
tcp_syn_probe.tcp_dst = opts[:port].to_i if opts[:port]
tcp_syn_probe.ip_daddr = opts[:ip] if opts[:ip]
return tcp_syn_probe
end
# Get a TCP ACK Probe Packet
def get_ack_probe()
tcp_ack_probe = get_tcp_packet()
tcp_ack_probe.tcp_flags.syn = 0
tcp_ack_probe.tcp_flags.ack = 1
return tcp_ack_probe
end
# Get a reflective TCP ACK Probe Packet from a TCP SYN/ACK Packet
# @param [PacketFu:TCPPacket] a syn/ack packet
def get_ack_from_syn_ack(syn_ack)
ack = get_ack_probe()
ack.ip_saddr = syn_ack.ip_daddr
ack.ip_daddr = syn_ack.ip_saddr
ack.eth_saddr = syn_ack.eth_daddr
ack.eth_daddr = syn_ack.eth_saddr
ack.tcp_sport = syn_ack.tcp_dport
ack.tcp_dport = syn_ack.tcp_sport
ack.tcp_ack = syn_ack.tcp_seq+1
ack.tcp_seq = syn_ack.tcp_ack
ack.tcp_win = 183
return ack
end
end
end
| {
"content_hash": "a9457d79e17af45dfbc8d093586b74ad",
"timestamp": "",
"source": "github",
"line_count": 69,
"max_line_length": 69,
"avg_line_length": 27.869565217391305,
"alnum_prop": 0.6177847113884556,
"repo_name": "claudijd/bnat",
"id": "10fd579f3f0f110751798c7a39c272db3923350c",
"size": "1923",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "lib/bnat/packet_factory.rb",
"mode": "33188",
"license": "bsd-3-clause",
"language": [
{
"name": "Ruby",
"bytes": "60410"
}
],
"symlink_target": ""
} |
angular.module('aa.shared')
.config(function($translateProvider) {
'use strict';
$translateProvider
.translations('en', {
'email_address': 'Email address',
'password': 'Password',
'password_repeat': 'Password repeat',
'your_password_contains': 'Your password contains',
'submit': 'Submit',
'characters_counter': '{charactersCount, plural, one{character} other{characters}}'
});
});
| {
"content_hash": "0b6d5c4986d3700d573a1fc7a94caf47",
"timestamp": "",
"source": "github",
"line_count": 15,
"max_line_length": 89,
"avg_line_length": 28.8,
"alnum_prop": 0.6412037037037037,
"repo_name": "ktor/training-advanced-angular",
"id": "7a96303a8fad8ebad64c734d62da3eb2dec1c3bb",
"size": "432",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "day1/src/app/_shared/translations/en-inline.js",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "HTML",
"bytes": "6283"
},
{
"name": "JavaScript",
"bytes": "26770"
}
],
"symlink_target": ""
} |
package com.example.android.sunshine.app;
/**
* Created by lmedina on 11/5/2015.
*/
/*
* Copyright (C) 2014 The Android Open Source Project
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import com.google.android.gms.common.api.ResultCallback;
import com.google.android.gms.wearable.DataApi;
import com.google.android.gms.wearable.DataItem;
import com.google.android.gms.wearable.DataMapItem;
public final class DigitalWatchFaceUtil {
private static final String TAG = "DigitalWatchFaceUtil";
public static final String PATH = "/sunshine_data_update";
public interface FetchConfigDataMapCallback {
void onConfigDataMapFetched(DataMapItem dataMapItem);
}
// private static int parseColor(String colorName) {
// return Color.parseColor(colorName.toLowerCase());
// }
//
// public static void fetchConfigDataMap(final GoogleApiClient client,
// final FetchConfigDataMapCallback callback) {
//// Wearable.NodeApi.getConnectedNodes(client);
//// Wearable.NodeApi.getLocalNode(client).setResultCallback(
//// new ResultCallback<NodeApi.GetLocalNodeResult>() {
//// @Override
//// public void onResult(NodeApi.GetLocalNodeResult getLocalNodeResult) {
//// String localNode = getLocalNodeResult.getNode().getId();
//// Uri uri = new Uri.Builder()
//// .scheme("wear")
//// .path(DigitalWatchFaceUtil.PATH)
//// .authority(localNode)
//// .build();
//// Wearable.DataApi.getDataItem(client, uri)
//// .setResultCallback(new DataItemResultCallback(callback));
//// }
//// }
//// );
// }
//
// /**
// * Overwrites (or sets, if not present) the keys in the current config {@link DataItem} with
// * the ones appearing in the given {@link DataMap}. If the config DataItem doesn't exist,
// * it's created.
// * <p>
// * It is allowed that only some of the keys used in the config DataItem appear in
// * {@code configKeysToOverwrite}. The rest of the keys remains unmodified in this case.
// */
//// public static void overwriteKeysInConfigDataMap(final GoogleApiClient googleApiClient,
//// final DataMap configKeysToOverwrite) {
////
//// DigitalWatchFaceUtil.fetchConfigDataMap(googleApiClient,
//// new FetchConfigDataMapCallback() {
//// @Override
//// public void onConfigDataMapFetched(DataMapItem dataMapItem) {
////// DataMap overwrittenConfig = new DataMap();
////// overwrittenConfig.putAll(dataMapItem.getDataMap());
////// overwrittenConfig.putAll(configKeysToOverwrite);
////
//// Log.e(TAG, "Max temp is " + configKeysToOverwrite.getString("maxTemp", "None"));
//// Log.e(TAG, "Min temp is " + configKeysToOverwrite.getString("minTemp", "None"));
//// Log.e(TAG, "Asset is " + configKeysToOverwrite.getAsset("weatherImage"));
////
//// Log.e(TAG, "DataMapFetched");
//// DigitalWatchFaceUtil.putConfigDataItem(googleApiClient, configKeysToOverwrite);
//// }
//// }
//// );
//// }
//
// /**
// * Overwrites the current config {@link DataItem}'s {@link DataMap} with {@code newConfig}.
// * If the config DataItem doesn't exist, it's created.
// */
// public static void putConfigDataItem(GoogleApiClient googleApiClient, DataMap newConfig) {
// PutDataMapRequest putDataMapRequest = PutDataMapRequest.create(PATH);
// DataMap configToPut = putDataMapRequest.getDataMap();
// configToPut.putAll(newConfig);
// Wearable.DataApi.putDataItem(googleApiClient, putDataMapRequest.asPutDataRequest())
// .setResultCallback(new ResultCallback<DataApi.DataItemResult>() {
// @Override
// public void onResult(DataApi.DataItemResult dataItemResult) {
// Log.e(TAG, "putDataItem result status: " + dataItemResult.getStatus());
// }
// });
// }
public static class DataItemResultCallback implements ResultCallback<DataApi.DataItemResult> {
private final FetchConfigDataMapCallback mCallback;
public DataItemResultCallback(FetchConfigDataMapCallback callback) {
mCallback = callback;
}
@Override
public void onResult(DataApi.DataItemResult dataItemResult) {
if (dataItemResult.getStatus().isSuccess()) {
if (dataItemResult.getDataItem() != null) {
DataItem configDataItem = dataItemResult.getDataItem();
DataMapItem dataMapItem = DataMapItem.fromDataItem(configDataItem);
mCallback.onConfigDataMapFetched(dataMapItem);
} else {
mCallback.onConfigDataMapFetched(null);
}
}
}
}
private DigitalWatchFaceUtil() { }
}
| {
"content_hash": "9a9afcac52a228c345ff8ea1d2dfc8ee",
"timestamp": "",
"source": "github",
"line_count": 131,
"max_line_length": 108,
"avg_line_length": 45.06106870229008,
"alnum_prop": 0.5974928002710487,
"repo_name": "Luis-Medina/Project6_AndroidWear",
"id": "0525eaca7529dc384fb49f9794e96d9eeb3859da",
"size": "5903",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "sunshinewear/src/main/java/com/example/android/sunshine/app/DigitalWatchFaceUtil.java",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "Java",
"bytes": "280180"
}
],
"symlink_target": ""
} |
def heard(data):
print "Speech Recognition Data:", data
lloyd = Runtime.createAndStart("lloyd", "ProgramAB")
lloyd.startSession("kevin", "alice2")
webgui = Runtime.create("WebGui","WebGui")
webgui.autoStartBrowser(False)
webgui.startService()
wksr = Runtime.createAndStart("webkitspeechrecognition", "WebkitSpeechRecognition")
htmlfilter = Runtime.createAndStart("htmlfilter", "HtmlFilter")
i01.mouth.speak("Testing to speak")
wksr.addTextListener(lloyd)
lloyd.addTextListener(htmlfilter)
htmlfilter.addTextListener(i01.mouth)
i01.mouth.speak("okay i am ready for conversation")
# and i used this from Alessandro
wksr.addListener("publishText","python","onText")
def onText(data):
print data
if (data == " let me take a picture of you"):
pose()
elif (data == "drive the car"):
drivecar()
| {
"content_hash": "a267038fdc6d71756bd46788380f86b4",
"timestamp": "",
"source": "github",
"line_count": 27,
"max_line_length": 83,
"avg_line_length": 30.703703703703702,
"alnum_prop": 0.7322074788902292,
"repo_name": "MyRobotLab/pyrobotlab",
"id": "a22200e766658f2608d3869df498b8886e60c88d",
"size": "855",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "home/Markus/webkitspeech.py",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "Batchfile",
"bytes": "1827"
},
{
"name": "C",
"bytes": "126258"
},
{
"name": "C++",
"bytes": "373018"
},
{
"name": "Java",
"bytes": "156911"
},
{
"name": "Processing",
"bytes": "17022"
},
{
"name": "Python",
"bytes": "3309101"
},
{
"name": "Shell",
"bytes": "4635"
},
{
"name": "VBA",
"bytes": "11115"
}
],
"symlink_target": ""
} |
<?php
/**
* @namespace
*/
namespace Zend\OpenId\Provider\User;
/**
* Abstract class to get/store information about logged in user in Web Browser
*
* @category Zend
* @package Zend_OpenId
* @subpackage Zend\OpenId\Provider\GenericProvider
* @copyright Copyright (c) 2005-2011 Zend Technologies USA Inc. (http://www.zend.com)
* @license http://framework.zend.com/license/new-bsd New BSD License
*/
abstract class AbstractUser
{
/**
* Stores information about logged in user
*
* @param string $id user identity URL
* @return bool
*/
abstract public function setLoggedInUser($id);
/**
* Returns identity URL of logged in user or false
*
* @return mixed
*/
abstract public function getLoggedInUser();
/**
* Performs logout. Clears information about logged in user.
*
* @return bool
*/
abstract public function delLoggedInUser();
}
| {
"content_hash": "ed5e3842d116fc50a671a49e359e642d",
"timestamp": "",
"source": "github",
"line_count": 43,
"max_line_length": 87,
"avg_line_length": 21.976744186046513,
"alnum_prop": 0.6476190476190476,
"repo_name": "phphatesme/LiveTest",
"id": "dfa2124782e5dc4f308503a48179dd9cf8a7d250",
"size": "1669",
"binary": false,
"copies": "5",
"ref": "refs/heads/master",
"path": "src/lib/Zend/OpenId/Provider/User/AbstractUser.php",
"mode": "33261",
"license": "mit",
"language": [
{
"name": "PHP",
"bytes": "14662639"
},
{
"name": "Shell",
"bytes": "613"
}
],
"symlink_target": ""
} |
using System;
using Alachisoft.NCache.Common.Pooling;
using Alachisoft.NCache.IO;
namespace Alachisoft.NCache.Serialization.Surrogates
{
/// <summary>
/// Surrogate for types that inherit from <see cref="ICompactSerializable"/>.
/// </summary>
public abstract class ContextSensitiveSerializationSurrogate : SerializationSurrogate
{
public ContextSensitiveSerializationSurrogate(Type t, IObjectPool pool) : base(t, pool) { }
abstract public object ReadDirect(CompactBinaryReader reader, object graph);
abstract public void WriteDirect(CompactBinaryWriter writer, object graph);
abstract public void SkipDirect(CompactBinaryReader reader, object graph);
virtual public object Instantiate(CompactBinaryReader reader)
{
return base.CreateInstance();
}
sealed public override object Read(CompactBinaryReader reader)
{
int cookie = reader.ReadInt32();
object graph = reader.Context.GetObject(cookie);
if (graph == null)
{
bool bKnown = false;
graph = Instantiate(reader);
if (graph != null)
{
reader.Context.RememberObject(graph, false);
bKnown = true;
}
if (VersionCompatible)
{
long startPosition = 0;
int dataLength = 0;
long endPosition = 0;
startPosition = reader.BaseReader.BaseStream.Position;
dataLength = reader.ReadInt32();
reader.BeginCurrentObjectDeserialization(startPosition + dataLength);
graph = ReadDirect(reader, graph);
reader.EndCurrentObjectDeserialization();
if (dataLength != -1 && (endPosition - startPosition) < dataLength)
{
endPosition = reader.BaseReader.BaseStream.Position;
reader.SkipBytes((int)(dataLength - (endPosition - startPosition)));
}
}
else
{
reader.BeginCurrentObjectDeserialization(reader.BaseReader.BaseStream.Length);
graph = ReadDirect(reader, graph);
reader.EndCurrentObjectDeserialization();
}
if (!bKnown)
{
reader.Context.RememberObject(graph, false);
}
}
return graph;
}
public override void Write(CompactBinaryWriter writer, object graph)
{
int cookie = writer.Context.GetCookie(graph);
if (cookie != SerializationContext.INVALID_COOKIE)
{
writer.Write(cookie);
return;
}
cookie = writer.Context.RememberObject(graph, true);
writer.Write(cookie);
if (VersionCompatible)
{
long startPosition = 0;
long endPosition = 0;
startPosition = writer.BaseWriter.BaseStream.Position;
writer.Write((int)0);
WriteDirect(writer, graph);
endPosition = writer.BaseWriter.BaseStream.Position;
writer.BaseWriter.BaseStream.Seek(startPosition, System.IO.SeekOrigin.Begin);
writer.Write((int)(endPosition - startPosition));
writer.BaseWriter.BaseStream.Seek(endPosition, System.IO.SeekOrigin.Begin);
}
else
{
WriteDirect(writer, graph);
}
}
sealed public override void Skip(CompactBinaryReader reader)
{
int cookie = reader.ReadInt32();
object graph = reader.Context.GetObject(cookie);
if (graph == null)
{
graph = Instantiate(reader);
SkipDirect(reader, graph);
}
}
}
} | {
"content_hash": "4b6b498880b9ba6aadce8e13cc561280",
"timestamp": "",
"source": "github",
"line_count": 115,
"max_line_length": 99,
"avg_line_length": 35.93043478260869,
"alnum_prop": 0.5336398838334947,
"repo_name": "Alachisoft/NCache",
"id": "f937caa91656710dd0e222ea97b33c5fa1101b0a",
"size": "4737",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "Src/NCSerialization/Surrogates/ContextSensitiveSerializationSurrogate.cs",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "C#",
"bytes": "12283536"
},
{
"name": "CSS",
"bytes": "707"
},
{
"name": "HTML",
"bytes": "16825"
},
{
"name": "JavaScript",
"bytes": "377"
}
],
"symlink_target": ""
} |
package org.cipres.treebase.dao.jdbc;
import org.apache.log4j.Logger;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.sql.Types;
import java.util.List;
import org.springframework.jdbc.UncategorizedSQLException;
/**
* MatrixElementJDBC.java
*
* Created on Jan 16, 2008
*
* @author Jin Ruan
*
*/
public class DiscreteMatrixElementJDBC {
private static final Logger LOGGER = Logger.getLogger(DiscreteMatrixElementJDBC.class);
// public static final char TYPE_COMPOUND = 'C';
// public static final char TYPE_CONINUOUS = 'N';
// public static final char TYPE_DISCRETE = 'D';
private short mGap = 0;
private long mDiscreteCharState_ID;
private long mMatrixColID;
private long mMatrixRowID;
private int mElementOrder;
// MATRIXELEMENT_ID, VERSION,
// MATRIXCOLUMN_ID, MATRIXROW_ID, ELEMENT_ORDER
// TYPE, COMPOUNDVALUE, ANDLOGIC,
// for compound element
// DISCRETECHARSTATE_ID, GAP
// for discrete state
// VALUE, ITEMDEFINITION_ID,
// Value is double, for continuous element
// ******************************************************/
// INSERT INTO MATRIXELEMENT(TYPE, MATRIXELEMENT_ID, VERSION,
// COMPOUNDVALUE, ANDLOGIC, VALUE, GAP, MATRIXCOLUMN_ID,
// ITEMDEFINITION_ID, MATRIXROW_ID, DISCRETECHARSTATE_ID, ELEMENT_ORDER)
// VALUES(?, default, 1,
// '', 0, 0, 0, 0,
// 0, 0, 0, 0)
/**
* Constructor.
*/
public DiscreteMatrixElementJDBC() {
super();
}
/**
*
* Creation date: Mar 20, 2008 10:24:56 AM
*/
public static void batchDiscreteElements(
List<DiscreteMatrixElementJDBC> pElements,
Connection pCon) {
StringBuffer queryBuf = new StringBuffer(
"INSERT INTO MATRIXELEMENT(TYPE, MATRIXELEMENT_ID, VERSION, ");
queryBuf
.append("GAP, MATRIXCOLUMN_ID, MATRIXROW_ID, DISCRETECHARSTATE_ID, ELEMENT_ORDER) ")
.append("VALUES('D', default, 1, ?, ?, ?, ?, ?)");
try {
PreparedStatement ps = pCon.prepareStatement(queryBuf.toString());
int elementSize = pElements.size();
int count = 0;
for (int i = 0; i < elementSize; i++) {
DiscreteMatrixElementJDBC e = pElements.get(i);
// TYPE: D
// MatrixElement_ID: default
// Version: 1
// // not set here:
// CompoundValue: '' (for compound)
// ANDLogic: 0 (for compound)
// Value: (continuous)
// ITEMDEFINITION_ID: (continuous)
ps.setBoolean(1, e.getGap()); // Gap
ps.setLong(2, e.getMatrixColID()); // MATRIXCOLUMN_ID
ps.setLong(3, e.getMatrixRowID()); // MATRIXROW_ID
if (e.getDiscreteCharState_ID() > 0) {
ps.setLong(4, e.getDiscreteCharState_ID()); // DISCRETECHARSTATE_ID
} else {
ps.setNull(4, Types.BIGINT);
}
ps.setInt(5, e.getElementOrder()); // ELEMENT_ORDER
ps.addBatch();
count++;
//IBM JDBC driver has a 32k batch limit:
if (count > MatrixJDBC.JDBC_BATCH_LIMIT) {
ps.executeBatch();
pCon.commit();
if (LOGGER.isDebugEnabled()) {
LOGGER.debug("committing count=" + count); //$NON-NLS-1$
}
count = 0;
}
}
ps.executeBatch();
pCon.commit();
ps.close();
if (LOGGER.isDebugEnabled()) {
LOGGER.debug("committing count=" + count); //$NON-NLS-1$
}
} catch (SQLException ex) {
throw new UncategorizedSQLException("Failed to batch discrete matrix elements.", "", ex);
}
}
/**
* Return the Gap field.
*
* @return short mGap
*/
public boolean getGap() {
return (mGap!=0);
}
/**
* Set the Gap field.
*/
public void setGap(short pNewGap) {
mGap = pNewGap;
}
/**
* Return the DiscreteCharState_ID field.
*
* @return long mDiscreteCharState_ID
*/
public long getDiscreteCharState_ID() {
return mDiscreteCharState_ID;
}
/**
* Set the DiscreteCharState_ID field.
*/
public void setDiscreteCharState_ID(long pNewDiscreteCharState_ID) {
mDiscreteCharState_ID = pNewDiscreteCharState_ID;
}
/**
* Return the MatrixRowID field.
*
* @return long mMatrixRowID
*/
public long getMatrixRowID() {
return mMatrixRowID;
}
/**
* Set the MatrixRowID field.
*/
public void setMatrixRowID(long pNewMatrixRowID) {
mMatrixRowID = pNewMatrixRowID;
}
/**
* Return the MatrixColID field.
*
* @return long mMatrixColID
*/
public long getMatrixColID() {
return mMatrixColID;
}
/**
* Set the MatrixColID field.
*/
public void setMatrixColID(long pNewMatrixColID) {
mMatrixColID = pNewMatrixColID;
}
/**
* Return the ElementOrder field.
*
* @return int mElementOrder
*/
public int getElementOrder() {
return mElementOrder;
}
/**
* Set the ElementOrder field.
*/
public void setElementOrder(int pNewElementOrder) {
mElementOrder = pNewElementOrder;
}
}
| {
"content_hash": "4fbe23634af537940d127fc6bb8c836c",
"timestamp": "",
"source": "github",
"line_count": 216,
"max_line_length": 92,
"avg_line_length": 22.89351851851852,
"alnum_prop": 0.627098078867543,
"repo_name": "TreeBASE/treebasetest",
"id": "89dec5e23cc541cf4713313373c52633970259ad",
"size": "4945",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "treebase-core/src/main/java/org/cipres/treebase/dao/jdbc/DiscreteMatrixElementJDBC.java",
"mode": "33188",
"license": "bsd-3-clause",
"language": [
{
"name": "ApacheConf",
"bytes": "172"
},
{
"name": "Batchfile",
"bytes": "62"
},
{
"name": "CSS",
"bytes": "39246"
},
{
"name": "HTML",
"bytes": "1330382"
},
{
"name": "Java",
"bytes": "3078667"
},
{
"name": "JavaScript",
"bytes": "107821"
},
{
"name": "PHP",
"bytes": "127834"
},
{
"name": "PLSQL",
"bytes": "653"
},
{
"name": "PLpgSQL",
"bytes": "31601"
},
{
"name": "Perl",
"bytes": "393086"
},
{
"name": "Perl6",
"bytes": "38254"
},
{
"name": "Shell",
"bytes": "9031"
},
{
"name": "Web Ontology Language",
"bytes": "7720"
}
],
"symlink_target": ""
} |
"use strict";
var _interopRequireDefault = require("@babel/runtime/helpers/interopRequireDefault");
var _interopRequireWildcard = require("@babel/runtime/helpers/interopRequireWildcard");
var _react = _interopRequireWildcard(require("react"));
var _ink = require("ink");
var _context = _interopRequireWildcard(require("./context"));
var _cli = _interopRequireDefault(require("./cli"));
const ConnectedCLI = () => {
const state = (0, _react.useContext)(_context.default);
const showStatusBar = state.program && state.program._ && state.program._[0] === `develop` && state.program.status === `BOOTSTRAP_FINISHED`;
return _react.default.createElement(_cli.default, {
showStatusBar: Boolean(showStatusBar),
logs: state.logs
});
};
(0, _ink.render)(_react.default.createElement(_context.StoreStateProvider, null, _react.default.createElement(ConnectedCLI, null))); | {
"content_hash": "dfc0a4f22cc804a9c32c782e3fd76b64",
"timestamp": "",
"source": "github",
"line_count": 24,
"max_line_length": 142,
"avg_line_length": 36.791666666666664,
"alnum_prop": 0.72480181200453,
"repo_name": "BigBoss424/portfolio",
"id": "4ba8da1c13c24fb93e1756d08c35f390622ba016",
"size": "883",
"binary": false,
"copies": "2",
"ref": "refs/heads/master",
"path": "v7/development/node_modules/gatsby/node_modules/gatsby-cli/lib/reporter/loggers/ink/index.js",
"mode": "33188",
"license": "apache-2.0",
"language": [],
"symlink_target": ""
} |
var app = require('app');
var BrowserWindow = require('browser-window');
var google = require('googleapis');
// Report crashes to our server.
require('crash-reporter').start();
// Keep a global reference of the window object, if you don't, the window will
// be closed automatically when the javascript object is GCed.
var mainWindow = null;
// Quit when all windows are closed.
app.on('window-all-closed', function(){
if(process.platform != 'darwin'){
app.quit();
}
});
// This method will be called when Electron has done everything
// initialization and ready for creating browser windows.
app.on('ready', function(){
// Create the browser window.
mainWindow = new BrowserWindow({width: 400, height: 600});
// and load the index.html of the app.
mainWindow.loadUrl('file://' + __dirname + '/app/index.html');
// Open the devtools.
// mainWindow.openDevTools();
// Emitted when the window is closed.
mainWindow.on('closed', function() {
// Dereference the window object, usually you would store windows
// in an array if your app supports multi windows, this is the time
// when you should delete the corresponding element.
mainWindow = null;
});
}); | {
"content_hash": "0c6c135f43d045fda8e89934b5cad1d5",
"timestamp": "",
"source": "github",
"line_count": 38,
"max_line_length": 78,
"avg_line_length": 31.026315789473685,
"alnum_prop": 0.7099236641221374,
"repo_name": "raks437/rHangout",
"id": "c8a2a1e85eeb84929990455ed7d768c83e9d0c4a",
"size": "1179",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "main.js",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "HTML",
"bytes": "927"
},
{
"name": "JavaScript",
"bytes": "1236"
}
],
"symlink_target": ""
} |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1">
<!-- The above 3 meta tags *must* come first in the head; any other head content must come *after* these tags -->
<title>Yêu Mãi</title>
<!-- Bootstrap -->
<link href="bootstrap/css/bootstrap.min.css" rel="stylesheet">
<link href="cusStyles.css" rel="stylesheet" />
<!-- HTML5 shim and Respond.js for IE8 support of HTML5 elements and media queries -->
<!-- WARNING: Respond.js doesn't work if you view the page via file:// -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/html5shiv/3.7.3/html5shiv.min.js"></script>
<script src="https://oss.maxcdn.com/respond/1.4.2/respond.min.js"></script>
<![endif]-->
</head>
<body>
<h1 class="text-primary">Yêu Mãi</h1>
<div class="container">
<div class="row">
<div class= "col-xs-14">
<form action="newuser.php" method="post">
<b>Add a New User </b>
<p>User Name:
<input type="text" name="UserName"size="25" maxlength="30" /></p>
<p>First Name:
<input type="text" name="FirstName" size="25" maxlength="30" /></p>
<p>Last Name:
<input type="text" name="LastName" size="25" maxlength="30" /></p>
<p>Email:
<input type="text" name="Email" size="25" maxlength="40" /></p>
<p>Living Area:
<input type="text" name="HomeAddress" size="25" maxlength="50" /></p>
<p>Home Phone:
<input type="text" name="HomePhone" size="25" maxlength="20" /></p>
<p>Cell Phone:
<input type="text" name="CellPhone" size="25" maxlength="20" /></p>
<p>Year of Birth:
<input type="text" name="YearBirth" size="25" maxlength="20" /></p>
<p>Female/Male:
<input type="text" name="Sex" size="25" maxlength="20" /></p>
<input type="submit" name="submit" value="Send" />
</form>
</div>
</div>
</div>
<!-- jQuery (necessary for Bootstrap's JavaScript plugins) -->
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.12.4/jquery.min.js"></script>
<!-- Include all compiled plugins (below), or include individual files as needed -->
<script src="bootstrap/js/bootstrap.min.js"></script>
</body>
</html>
| {
"content_hash": "c7ca0df04c53f8ee045391ab8107fdee",
"timestamp": "",
"source": "github",
"line_count": 58,
"max_line_length": 117,
"avg_line_length": 40.241379310344826,
"alnum_prop": 0.6066838046272494,
"repo_name": "phongdong/match-all",
"id": "afb41e9ba0d20718aebb8face3dcb0aff135bda3",
"size": "2338",
"binary": false,
"copies": "2",
"ref": "refs/heads/master",
"path": "yeumai/createuser.php",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "CSS",
"bytes": "2791"
},
{
"name": "JavaScript",
"bytes": "11264"
},
{
"name": "PHP",
"bytes": "177741"
}
],
"symlink_target": ""
} |
package com.vmware.vim25;
/**
@author Steve Jin ([email protected])
*/
public class PatchInstallFailed extends PlatformConfigFault
{
public boolean rolledBack;
public boolean isRolledBack()
{
return this.rolledBack;
}
public void setRolledBack(boolean rolledBack)
{
this.rolledBack=rolledBack;
}
} | {
"content_hash": "34851debd3833ba8b97983df3c462eaf",
"timestamp": "",
"source": "github",
"line_count": 22,
"max_line_length": 59,
"avg_line_length": 14.681818181818182,
"alnum_prop": 0.7275541795665634,
"repo_name": "mikem2005/vijava",
"id": "f7113678557729e70a7cf2715504f2dc97f97ae5",
"size": "1965",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "src/com/vmware/vim25/PatchInstallFailed.java",
"mode": "33188",
"license": "bsd-3-clause",
"language": [
{
"name": "Java",
"bytes": "6585830"
}
],
"symlink_target": ""
} |
import os
import rediswq
from model_training import FraudDetectionModelTrainer
# Initialize variables
FILESTORE_PATH = "/mnt/fileserver/"
TESTING_DATASET_PATH = FILESTORE_PATH + "datasets/testing/test_dataset.pkl"
OUTPUT_DIR = FILESTORE_PATH + "output/"
REPORT_PATH = OUTPUT_DIR + "report.txt"
CLASS_LABEL = "TX_FRAUD_SCENARIO"
QUEUE_NAME = "datasets"
HOST = "redis"
def main():
"""
Workload which:
1. Claims a filename from a Redis Worker Queue
2. Reads the dataset from the file
3. Partially trains the model on the dataset
4. Saves a model checkpoint and generates a report on
the performance of the model after the partial training.
5. Removes the filename from the Redis Worker Queue
6. Repeats 1 through 5 till the Queue is empty
"""
q = rediswq.RedisWQ(name="datasets", host=HOST)
print("Worker with sessionID: " + q.sessionID())
print("Initial queue state: empty=" + str(q.empty()))
checkpoint_path = None
while not q.empty():
# Claim item in Redis Worker Queue
item = q.lease(lease_secs=20, block=True, timeout=2)
if item is not None:
dataset_path = item.decode("utf-8")
print("Processing dataset: " + dataset_path)
training_dataset_path = FILESTORE_PATH + dataset_path
# Initialize the model training manager class
model_trainer = FraudDetectionModelTrainer(
training_dataset_path,
TESTING_DATASET_PATH,
CLASS_LABEL,
checkpoint_path=checkpoint_path,
)
# Train model and save checkpoint + report
checkpoint_path = model_trainer.train_and_save(OUTPUT_DIR)
model_trainer.generate_report(REPORT_PATH)
# Remove item from Redis Worker Queue
q.complete(item)
else:
print("Waiting for work")
print("Queue empty, exiting")
# Run workload
main()
| {
"content_hash": "544e01c1de57bd485ee1b2a6c5d2b53c",
"timestamp": "",
"source": "github",
"line_count": 58,
"max_line_length": 75,
"avg_line_length": 34.05172413793103,
"alnum_prop": 0.6369620253164557,
"repo_name": "GoogleCloudPlatform/kubernetes-engine-samples",
"id": "16286cdef198b8976dd3a33ab2c32c035f538f8d",
"size": "2573",
"binary": false,
"copies": "1",
"ref": "refs/heads/main",
"path": "batch/aiml-workloads/src/worker.py",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "C#",
"bytes": "2726"
},
{
"name": "Dockerfile",
"bytes": "17562"
},
{
"name": "Go",
"bytes": "25649"
},
{
"name": "HCL",
"bytes": "6779"
},
{
"name": "HTML",
"bytes": "2856"
},
{
"name": "Java",
"bytes": "675"
},
{
"name": "JavaScript",
"bytes": "1914"
},
{
"name": "PHP",
"bytes": "1594"
},
{
"name": "Procfile",
"bytes": "18"
},
{
"name": "Python",
"bytes": "29836"
},
{
"name": "Ruby",
"bytes": "216"
},
{
"name": "Shell",
"bytes": "13206"
}
],
"symlink_target": ""
} |
module Mokio
class SeoTag < ActiveRecord::Base
include Mokio::Concerns::Models::SeoTag
end
end | {
"content_hash": "3e5c885cf9bddb1cc2de4423556d24b2",
"timestamp": "",
"source": "github",
"line_count": 5,
"max_line_length": 43,
"avg_line_length": 20.4,
"alnum_prop": 0.7450980392156863,
"repo_name": "versoft/mokio",
"id": "1593999c811f487ee68801a6b3adb6a5822aca68",
"size": "102",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "app/models/mokio/seo_tag.rb",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "CSS",
"bytes": "123619"
},
{
"name": "CoffeeScript",
"bytes": "1784"
},
{
"name": "HTML",
"bytes": "10813"
},
{
"name": "Haml",
"bytes": "9434"
},
{
"name": "JavaScript",
"bytes": "1387750"
},
{
"name": "Ruby",
"bytes": "489995"
},
{
"name": "SCSS",
"bytes": "299517"
},
{
"name": "Shell",
"bytes": "13381"
},
{
"name": "Slim",
"bytes": "83766"
}
],
"symlink_target": ""
} |
'''
Licensed to the Apache Software Foundation (ASF) under one
or more contributor license agreements. See the NOTICE file
distributed with this work for additional information
regarding copyright ownership. The ASF licenses this file
to you under the Apache License, Version 2.0 (the
"License"); you may not use this file except in compliance
with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
'''
import json
from mock.mock import MagicMock, call, patch
from stacks.utils.RMFTestCase import *
import resource_management.core.source
from test_storm_base import TestStormBase
class TestStormNimbus(TestStormBase):
def test_configure_default(self):
self.executeScript(self.COMMON_SERVICES_PACKAGE_DIR + "/scripts/nimbus_prod.py",
classname = "Nimbus",
command = "configure",
config_file="default.json",
stack_version = self.STACK_VERSION,
target = RMFTestCase.TARGET_COMMON_SERVICES
)
self.assert_configure_default()
self.assertNoMoreResources()
def test_start_default(self):
self.executeScript(self.COMMON_SERVICES_PACKAGE_DIR + "/scripts/nimbus_prod.py",
classname = "Nimbus",
command = "start",
config_file="default.json",
stack_version = self.STACK_VERSION,
target = RMFTestCase.TARGET_COMMON_SERVICES
)
self.assert_configure_default()
self.assertResourceCalled('Execute', 'supervisorctl start storm-nimbus',
wait_for_finish = False,
)
self.assertNoMoreResources()
@patch("os.path.exists")
def test_stop_default(self, path_exists_mock):
# Last bool is for the pid file
path_exists_mock.side_effect = [False, False, True]
self.executeScript(self.COMMON_SERVICES_PACKAGE_DIR + "/scripts/nimbus_prod.py",
classname = "Nimbus",
command = "stop",
config_file="default.json",
stack_version = self.STACK_VERSION,
target = RMFTestCase.TARGET_COMMON_SERVICES
)
self.assertResourceCalled('Execute', 'supervisorctl stop storm-nimbus',
wait_for_finish = False,
)
self.assertNoMoreResources()
def test_configure_secured(self):
self.executeScript(self.COMMON_SERVICES_PACKAGE_DIR + "/scripts/nimbus_prod.py",
classname = "Nimbus",
command = "configure",
config_file="secured.json",
stack_version = self.STACK_VERSION,
target = RMFTestCase.TARGET_COMMON_SERVICES
)
self.assert_configure_secured()
self.assertNoMoreResources()
def test_start_secured(self):
self.executeScript(self.COMMON_SERVICES_PACKAGE_DIR + "/scripts/nimbus_prod.py",
classname = "Nimbus",
command = "start",
config_file="secured.json",
stack_version = self.STACK_VERSION,
target = RMFTestCase.TARGET_COMMON_SERVICES
)
self.assert_configure_secured()
self.assertResourceCalled('Execute', 'supervisorctl start storm-nimbus',
wait_for_finish = False,
)
self.assertNoMoreResources()
@patch("os.path.exists")
def test_stop_secured(self, path_exists_mock):
# Last bool is for the pid file
path_exists_mock.side_effect = [False, False, True]
self.executeScript(self.COMMON_SERVICES_PACKAGE_DIR + "/scripts/nimbus_prod.py",
classname = "Nimbus",
command = "stop",
config_file="secured.json",
stack_version = self.STACK_VERSION,
target = RMFTestCase.TARGET_COMMON_SERVICES
)
self.assertResourceCalled('Execute', 'supervisorctl stop storm-nimbus',
wait_for_finish = False,
)
self.assertNoMoreResources()
def test_pre_upgrade_restart(self):
self.executeScript(self.COMMON_SERVICES_PACKAGE_DIR + "/scripts/nimbus_prod.py",
classname = "Nimbus",
command = "pre_upgrade_restart",
config_file="default.json",
stack_version = self.STACK_VERSION,
target = RMFTestCase.TARGET_COMMON_SERVICES)
self.assertResourceCalled("Execute", ('ambari-python-wrap', '/usr/bin/hdp-select', 'set', 'storm-client', '2.2.1.0-2067'), sudo=True)
self.assertResourceCalled("Execute", ('ambari-python-wrap', '/usr/bin/hdp-select', 'set', 'storm-nimbus', '2.2.1.0-2067'), sudo=True)
self.assertNoMoreResources()
def test_pre_upgrade_restart_23(self):
config_file = self.get_src_folder()+"/test/python/stacks/2.1/configs/default.json"
with open(config_file, "r") as f:
json_content = json.load(f)
version = '2.3.0.0-1234'
json_content['commandParams']['version'] = version
mocks_dict = {}
self.executeScript(self.COMMON_SERVICES_PACKAGE_DIR + "/scripts/nimbus_prod.py",
classname = "Nimbus",
command = "pre_upgrade_restart",
config_dict = json_content,
stack_version = self.STACK_VERSION,
target = RMFTestCase.TARGET_COMMON_SERVICES,
call_mocks = [(0, None, ''), (0, None, '')],
mocks_dict = mocks_dict)
self.assertResourceCalledIgnoreEarlier("Execute", ('ambari-python-wrap', '/usr/bin/hdp-select', 'set', 'storm-client', '2.3.0.0-1234'), sudo=True)
self.assertResourceCalled("Execute", ('ambari-python-wrap', '/usr/bin/hdp-select', 'set', 'storm-nimbus', '2.3.0.0-1234'), sudo=True)
self.assertEquals(1, mocks_dict['call'].call_count)
self.assertEquals(1, mocks_dict['checked_call'].call_count)
self.assertEquals(
('ambari-python-wrap', '/usr/bin/conf-select', 'set-conf-dir', '--package', 'storm', '--stack-version', '2.3.0.0-1234', '--conf-version', '0'),
mocks_dict['checked_call'].call_args_list[0][0][0])
self.assertEquals(
('ambari-python-wrap', '/usr/bin/conf-select', 'create-conf-dir', '--package', 'storm', '--stack-version', '2.3.0.0-1234', '--conf-version', '0'),
mocks_dict['call'].call_args_list[0][0][0])
self.assertNoMoreResources()
| {
"content_hash": "f166a8d20e95f8716325ec56608bdcdd",
"timestamp": "",
"source": "github",
"line_count": 153,
"max_line_length": 152,
"avg_line_length": 44.88235294117647,
"alnum_prop": 0.6076889471384884,
"repo_name": "alexryndin/ambari",
"id": "850a98bfee3e09dd651e1a10ffe5f1233baebe97",
"size": "6890",
"binary": false,
"copies": "1",
"ref": "refs/heads/branch-adh-1.5",
"path": "ambari-server/src/test/python/stacks/2.1/STORM/test_storm_nimbus_prod.py",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "Batchfile",
"bytes": "44884"
},
{
"name": "C",
"bytes": "331204"
},
{
"name": "C#",
"bytes": "215907"
},
{
"name": "C++",
"bytes": "257"
},
{
"name": "CSS",
"bytes": "786184"
},
{
"name": "CoffeeScript",
"bytes": "8465"
},
{
"name": "FreeMarker",
"bytes": "2654"
},
{
"name": "Groovy",
"bytes": "89958"
},
{
"name": "HTML",
"bytes": "2514774"
},
{
"name": "Java",
"bytes": "29565801"
},
{
"name": "JavaScript",
"bytes": "19033151"
},
{
"name": "Makefile",
"bytes": "11111"
},
{
"name": "PHP",
"bytes": "149648"
},
{
"name": "PLpgSQL",
"bytes": "316489"
},
{
"name": "PowerShell",
"bytes": "2090340"
},
{
"name": "Python",
"bytes": "17215686"
},
{
"name": "R",
"bytes": "3943"
},
{
"name": "Roff",
"bytes": "13935"
},
{
"name": "Ruby",
"bytes": "33764"
},
{
"name": "SQLPL",
"bytes": "4277"
},
{
"name": "Shell",
"bytes": "886011"
},
{
"name": "Vim script",
"bytes": "5813"
},
{
"name": "sed",
"bytes": "2303"
}
],
"symlink_target": ""
} |
'use strict';
/**
* Module dependencies.
*/
var mongoose = require('mongoose'),
Schema = mongoose.Schema,
crypto = require('crypto');
/**
* A Validation function for local strategy properties
*/
var validateLocalStrategyProperty = function(property) {
return ((this.provider !== 'local' && !this.updated) || property.length);
};
/**
* A Validation function for local strategy password
*/
var validateLocalStrategyPassword = function(password) {
return (this.provider !== 'local' || (password && password.length > 6));
};
/**
* User Schema
*/
var UserSchema = new Schema({
firstName: {
type: String,
trim: true,
default: '',
validate: [validateLocalStrategyProperty, 'Please fill in your first name']
},
lastName: {
type: String,
trim: true,
default: '',
validate: [validateLocalStrategyProperty, 'Please fill in your last name']
},
displayName: {
type: String,
trim: true
},
email: {
type: String,
trim: true,
default: '',
validate: [validateLocalStrategyProperty, 'Please fill in your email'],
match: [/.+\@.+\..+/, 'Please fill a valid email address']
},
username: {
type: String,
unique: 'testing error message',
required: 'Please fill in a username',
trim: true
},
password: {
type: String,
default: '',
validate: [validateLocalStrategyPassword, 'Password should be longer']
},
salt: {
type: String
},
provider: {
type: String,
required: 'Provider is required'
},
providerData: {},
additionalProvidersData: {},
roles: {
type: [{
type: String,
enum: ['user', 'admin']
}],
default: ['user']
},
birthDate: {
type: Date,
required: 'Please provide a birth date'
},
updated: {
type: Date
},
created: {
type: Date,
default: Date.now
},
/* For reset password */
resetPasswordToken: {
type: String
},
resetPasswordExpires: {
type: Date
},
journals: [{
startDate: {
type: Date
},
endDate: {
type: Date
},
entry: {
type: String
}
}],
meditationSettings: {
prep: {
type: Number,
default: 10
},
meditation: {
type: Number,
default: 600
},
relax: {
type: Number,
default: 150
}
}
});
/**
* Hook a pre save method to hash the password
*/
UserSchema.pre('save', function(next) {
this.salt = new Buffer(crypto.randomBytes(16).toString('base64'), 'base64');
this.password = this.hashPassword(this.password);
next();
});
/**
* Create instance method for hashing a password
*/
UserSchema.methods.hashPassword = function(password) {
if (this.salt && password) {
return crypto.pbkdf2Sync(password, this.salt, 10000, 64).toString('base64');
} else {
return password;
}
};
/**
* Create instance method for authenticating user
*/
UserSchema.methods.authenticate = function(password) {
return this.password === this.hashPassword(password);
};
/**
* Find possible not used username
*/
UserSchema.statics.findUniqueUsername = function(username, suffix, callback) {
var _this = this;
var possibleUsername = username + (suffix || '');
_this.findOne({
username: possibleUsername
}, function(err, user) {
if (!err) {
if (!user) {
callback(possibleUsername);
} else {
return _this.findUniqueUsername(username, (suffix || 0) + 1, callback);
}
} else {
callback(null);
}
});
};
mongoose.model('User', UserSchema);
| {
"content_hash": "ebc9f839e9a77861a77c5aa0ed362433",
"timestamp": "",
"source": "github",
"line_count": 173,
"max_line_length": 78,
"avg_line_length": 19.190751445086704,
"alnum_prop": 0.6469879518072289,
"repo_name": "ryanspillsbury90/HaleMeditates",
"id": "676e4180c9ecda9aac56fc676ad286c789ee7cdf",
"size": "3320",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "app/models/user.server.model.js",
"mode": "33261",
"license": "mit",
"language": [
{
"name": "CSS",
"bytes": "731"
},
{
"name": "HTML",
"bytes": "22942"
},
{
"name": "JavaScript",
"bytes": "86793"
},
{
"name": "Objective-C",
"bytes": "806"
},
{
"name": "Ruby",
"bytes": "293"
},
{
"name": "Shell",
"bytes": "17132"
},
{
"name": "Swift",
"bytes": "264356"
}
],
"symlink_target": ""
} |
<div class="row">
<form id="loginForm" action="login" class="col-md-8 col-md-offset-2" onsubmit="checkIframe(); return false">
<div>
<p id="loginMessage" />
</div>
<div class="form-group">
<input id="username" type="text" class="form-control input-lg" placeholder="Email">
</div>
<div class="form-group">
<input id="password" type="password" class="form-control input-lg" placeholder="Password">
</div>
<div class="form-group">
<button class="btn btn-primary btn-lg btn-block">Ingresar</button>
</div>
</form>
</div> | {
"content_hash": "519511d0bcf10561de094227a6c95118",
"timestamp": "",
"source": "github",
"line_count": 19,
"max_line_length": 110,
"avg_line_length": 30.473684210526315,
"alnum_prop": 0.6217616580310881,
"repo_name": "Wirwing/play-chat",
"id": "5d16badbc8af8cab3ad8b2b95d8861f82b77f901",
"size": "579",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "public/javascripts/login.js",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "CSS",
"bytes": "818"
},
{
"name": "JavaScript",
"bytes": "667"
},
{
"name": "Scala",
"bytes": "5993"
},
{
"name": "Shell",
"bytes": "16567"
}
],
"symlink_target": ""
} |
var slideshow;
var slides;
var lightboxTarget;
var notes;
var slideIndicator;
var timerStart;
var bookmarks = [];
$.ready().then(function(){
var FoveaSlideshow = document.registerElement('fovea-slideshow');
slideshow = $('fovea-slideshow');
slides = $$('slide');
notes = $$('notes');
slideshow.setAttribute('black', true);
window.addEventListener('resize', resizeSlides);
Mousetrap.bind(['right', 'down', 'pagedown', 'space', 'enter'], function() { updateSlide('next') });
Mousetrap.bind(['left', 'up', 'pageup'], function() { updateSlide('previous') });
Mousetrap.bind('b', function() { hideSlideshow() });
Mousetrap.bind('n', function() { toggleNotes() });
Mousetrap.bind('a', function() { resetSaturationScreen() });
Mousetrap.bind('s', function() { saturateScreen() });
Mousetrap.bind('d', function() { superSaturateScreen() });
Mousetrap.bind('c', function() { toggleClock() });
Mousetrap.bind('t', function() { toggleTimer() });
Mousetrap.bind('home', function() { updateSlide(0) });
Mousetrap.bind('end', function() { updateSlide(-1) });
Mousetrap.bind('1', function() { goToBookmark(1) });
Mousetrap.bind('2', function() { goToBookmark(2) });
Mousetrap.bind('3', function() { goToBookmark(3) });
Mousetrap.bind('4', function() { goToBookmark(4) });
Mousetrap.bind('5', function() { goToBookmark(5) });
Mousetrap.bind('6', function() { goToBookmark(6) });
Mousetrap.bind('7', function() { goToBookmark(7) });
Mousetrap.bind('8', function() { goToBookmark(8) });
Mousetrap.bind('9', function() { goToBookmark(9) });
Mousetrap.bind('0', function() { updateSlide(0) });
initSlideshow();
})
function initSlideshow() {
resizeSlides();
prepareSlides();
createSlideIndicator();
prepareZoomableImages();
createClock();
createTimer();
window.setTimeout(function(){
updateSlide('start');
}, 200);
}
function updateSlide(method) {
var n = slideshow.getAttribute('currentSlide');
if(method=='start') {
var startState = History.getState();
if(startState.data.slide) {
var startSlide = startState.data.slide
} else {
var startSlide = slideshow.getAttribute('startSlide');
}
slideshow._.set({
attributes: {
'currentSlide': startSlide,
'black': false,
'saturate': false,
'superSaturate': false,
'shownotes': false
}
});
}
if(method=='next') {
if(n<slides.length) slideshow.setAttribute('currentslide', parseInt(slideshow.getAttribute('currentslide'))+1);
}
if(method=='previous') {
if(n>1) slideshow.setAttribute('currentslide', parseInt(slideshow.getAttribute('currentslide'))-1);
}
if(isInt(method)) {
slideshow.setAttribute('currentslide', parseInt(method)+1);
if(parseInt(method)==-1) {
slideshow.setAttribute('currentslide', slides.length);
}
}
n = slideshow.getAttribute('currentslide');
slides._.set({
attributes: {
visible: 'false',
focus: 'false'
}
});
if(n>1){
slides[n-2]._.set({
attributes: {
visible: 'true',
focus: 'false'
}
});
}
slides[n-1]._.set({
attributes: {
visible: 'true',
focus: 'true'
}
});
slideIndicator.style.width = ((n-1)/(slides.length-1))*100+'%';
History.pushState({'slide': n},'Slide '+n,'?slide='+n);
}
function goToBookmark(n) {
if(bookmarks[n-1]) {
updateSlide(bookmarks[n-1]);
}
}
function hideSlideshow() {
slideshow.setAttribute('black', !parseBoolean(slideshow.getAttribute('black')));
}
function resetSaturationScreen() {
slideshow.setAttribute('saturate', false);
slideshow.setAttribute('superSaturate', false);
}
function saturateScreen() {
slideshow.setAttribute('saturate', !parseBoolean(slideshow.getAttribute('saturate')));
}
function superSaturateScreen() {
slideshow.setAttribute('superSaturate', !parseBoolean(slideshow.getAttribute('superSaturate')));
}
function toggleNotes() {
slideshow.setAttribute('shownotes', !parseBoolean(slideshow.getAttribute('shownotes')));
}
function toggleClock() {
$('.clock').setAttribute('visible', !parseBoolean($('.clock').getAttribute('visible')));
}
function toggleTimer() {
$('.timer').setAttribute('visible', !parseBoolean($('.timer').getAttribute('visible')));
}
function resizeSlides() {
var body = $('body');
var wbox = body.getBoundingClientRect();
var ww = wbox.width;
var wh = wbox.height;
var wr = ww/wh;
var ssr = slideshow.getAttribute('ratio');
var ssbox = slideshow.getBoundingClientRect();
if(parseInt(ssr*100)<=parseInt(wr*100)) {
// alert('too wide ('+sr+', '+wr+')');
slideshow._.style({
width: ssbox.height*ssr+'px',
height: '100%'
});
}
else {
slideshow._.style({
height: ssbox.width/ssr+'px',
width: '100%'
});
}
}
function prepareSlides() {
slides.forEach(function(s, i){
if(s.getAttribute('background-image')) {
var bgSat = s.getAttribute('background-saturate')!=null ? s.getAttribute('background-saturate') : 1;
var bgBri = s.getAttribute('background-brightness')!=null ? s.getAttribute('background-brightness') : 1;
if(s.getAttribute('background-bw')){
bgSat = 0;
}
var bgSep = s.getAttribute('background-sepia') | 0;
var bgBlur = s.getAttribute('background-blur') | 0;
var bgFilter = s.getAttribute('background-cssgram');
var slideBackground = $.create("div", {
className: 'slideBackground',
style: {
backgroundImage: 'url('+ s.getAttribute('background-image')+')',
webkitFilter: 'saturate('+bgSat+') sepia('+bgSep+') brightness('+bgBri+') blur('+bgBlur+'px)',
filter: 'saturate('+bgSat+') sepia('+bgSep+') brightness('+bgBri+') blur('+bgBlur+'px)'
}
});
if(bgFilter!==null && bgFilter!=="")
slideBackground.classList.add(bgFilter);
s.appendChild(slideBackground);
}
if(s.getAttribute('bookmark')) {
bookmarks[s.getAttribute('bookmark')-1] = i;
}
});
}
function createSlideIndicator() {
var slideIndicatorCreator = $.create("div", {
className: "slideIndicator",
contents: [
{
tag: "div"
}
]
});
slideshow.appendChild(slideIndicatorCreator);
slideIndicator = $('.slideIndicator div');
}
function prepareZoomableImages() {
$$('[canzoom]')._.set({
attributes: {
'canzoom': true,
'iszoom': false
}
});
var lightboxCreator = $.create("div", {
className: "lightbox",
attributes: {
'iszoom': false
},
contents: [
{
tag: "img"
}
]
});
slideshow.appendChild(lightboxCreator);
$$('[canzoom]').forEach(function(el) {
el.addEventListener('click', zoomImage);
});
$('.lightbox').addEventListener('click', closeImage);
}
function zoomImage(e) {
console.log(e);
var img = $('.lightbox img');
var lightboxBox = $('.lightbox').getBoundingClientRect();
lightboxTarget = $(e.target);
var targetBoxSmall = lightboxTarget.getBoundingClientRect();
console.log(targetBoxSmall);
img._.set({
attributes: {
src: lightboxTarget.getAttribute('src'),
animate: false
},
style: {
position: 'relative',
width: '70%'
}
});
var targetBoxLarge = img.getBoundingClientRect();
img._.set({
attributes: {
visible: true,
top_s: targetBoxSmall.top-lightboxBox.top+'px',
left_s: targetBoxSmall.left-lightboxBox.left+'px',
right_s: targetBoxSmall.right-lightboxBox.right+'px',
bottom_s: targetBoxSmall.bottom-lightboxBox.bottom+'px',
width_s: targetBoxSmall.width+'px',
height_s: targetBoxSmall.height+'px',
top_l: targetBoxLarge.top-lightboxBox.top+'px',
left_l: targetBoxLarge.left-lightboxBox.left+'px',
right_l: targetBoxLarge.right-lightboxBox.right+'px',
bottom_l: targetBoxLarge.bottom-lightboxBox.bottom+'px',
width_l: targetBoxLarge.width+'px',
height_l: targetBoxLarge.height+'px'
},
style: {
position: 'fixed',
top: targetBoxSmall.top-lightboxBox.top+'px',
left: targetBoxSmall.left-lightboxBox.left+'px',
right: targetBoxSmall.right-lightboxBox.right+'px',
bottom: targetBoxSmall.bottom-lightboxBox.bottom+'px',
width: targetBoxSmall.width+'px',
height: targetBoxSmall.height+'px'
}
});
window.setTimeout(function() {
$('.lightbox').setAttribute('iszoom', true);
lightboxTarget.parentNode.setAttribute('zoomhide', true);
img._.set({
attributes: {
animate: true
},
style: {
top: targetBoxLarge.top-lightboxBox.top+'px',
left: targetBoxLarge.left-lightboxBox.left+'px',
right: targetBoxLarge.right-lightboxBox.right+'px',
bottom: targetBoxLarge.bottom-lightboxBox.bottom+'px',
width: targetBoxLarge.width+'px',
height: targetBoxLarge.height+'px'
}
});
}, 50);
}
function closeImage(e) {
var img = $('.lightbox img');
$('.lightbox').setAttribute('iszoom', false);
img._.set({
style: {
top: img.getAttribute('top_s'),
left: img.getAttribute('left_s'),
right: img.getAttribute('right_s'),
bottom: img.getAttribute('bottom_s'),
width: img.getAttribute('width_s'),
height: img.getAttribute('height_s')
}
});
window.setTimeout(function(){
img._.set({
attributes: {
animate: false,
visible: false
},
style: {
top: '',
left: '',
right: '',
bottom: '',
width: '',
height: ''
}
});
lightboxTarget.parentNode.setAttribute('zoomhide', false);
}, 450);
}
function createClock() {
var clockCreator = $.create("div", {
className: "clock",
attributes: {
'visible': false
},
contents: [
{
tag: "p"
}
]
});
slideshow.appendChild(clockCreator);
}
function createTimer() {
var timerCreator = $.create("div", {
className: "timer",
attributes: {
'visible': false
},
contents: [
{
tag: "p"
}
]
});
slideshow.appendChild(timerCreator);
timerStart = moment();
startTime();
}
function startTime() {
var now = new moment();
$('.clock p').innerHTML = now.format("HH:mm:ss");
var timer = now.valueOf() - timerStart.valueOf();
// var timer = moment.duration(timerMS);
$('.timer p').innerHTML = moment.utc(timer).format("HH:mm:ss");
var t = setTimeout(startTime, 500);
}
window.parseBoolean = function(string) {
var bool;
bool = (function() {
switch (false) {
case string.toLowerCase() !== 'true':
return true;
case string.toLowerCase() !== 'false':
return false;
}
})();
if (typeof bool === "boolean") {
return bool;
}
return void 0;
};
function isInt(value) {
var x;
if (isNaN(value)) {
return false;
}
x = parseFloat(value);
return (x | 0) === x;
}
| {
"content_hash": "b1eeb25d3dbe5b1757f58d85331d262d",
"timestamp": "",
"source": "github",
"line_count": 422,
"max_line_length": 115,
"avg_line_length": 25.98341232227488,
"alnum_prop": 0.6142270861833106,
"repo_name": "YarmoM/FoveaJS",
"id": "1f4215d6c898ce5ed768f80040423bba3e5d34fa",
"size": "10965",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "fovea.js",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "CSS",
"bytes": "16335"
},
{
"name": "JavaScript",
"bytes": "10965"
}
],
"symlink_target": ""
} |
SYNONYM
#### According to
The Catalogue of Life, 3rd January 2011
#### Published in
null
#### Original name
null
### Remarks
null | {
"content_hash": "96ec49888da01de703211409bd9c090b",
"timestamp": "",
"source": "github",
"line_count": 13,
"max_line_length": 39,
"avg_line_length": 10.23076923076923,
"alnum_prop": 0.6917293233082706,
"repo_name": "mdoering/backbone",
"id": "a67504c78666d7673e4ed97bd989b1839713d368",
"size": "194",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "life/Plantae/Magnoliophyta/Magnoliopsida/Rosales/Rosaceae/Rhaphiolepis/Rhaphiolepis indica/ Syn. Rhaphiolepis indica typica/README.md",
"mode": "33188",
"license": "apache-2.0",
"language": [],
"symlink_target": ""
} |
<?php
$GLOBALS['_scb_data'] = array( 60, __FILE__, array(
'scbUtil',
'scbOptions',
'scbForms',
'scbTable',
'scbWidget',
'scbAdminPage',
'scbBoxesPage',
'scbPostMetabox',
'scbCron',
'scbHooks',
) );
if ( ! class_exists( 'scbLoad4' ) ) :
/**
* The main idea behind this class is to load the most recent version of the scb classes available.
*
* It waits until all plugins are loaded and then does some crazy hacks to make activation hooks work.
*/
class scbLoad4 {
private static $candidates = array();
private static $classes;
private static $callbacks = array();
private static $loaded;
static function init( $callback = '' ) {
list( $rev, $file, $classes ) = $GLOBALS['_scb_data'];
self::$candidates[ $file ] = $rev;
self::$classes[ $file ] = $classes;
if ( ! empty( $callback ) ) {
self::$callbacks[ $file ] = $callback;
add_action( 'activate_plugin', array( __CLASS__, 'delayed_activation' ) );
}
if ( did_action( 'plugins_loaded' ) ) {
self::load();
} else {
add_action( 'plugins_loaded', array( __CLASS__, 'load' ), 9, 0 );
}
}
public static function delayed_activation( $plugin ) {
$plugin_dir = dirname( $plugin );
if ( '.' == $plugin_dir ) {
return;
}
foreach ( self::$callbacks as $file => $callback ) {
if ( dirname( dirname( plugin_basename( $file ) ) ) == $plugin_dir ) {
self::load( false );
call_user_func( $callback );
do_action( 'scb_activation_' . $plugin );
break;
}
}
}
public static function load( $do_callbacks = true ) {
arsort( self::$candidates );
$file = key( self::$candidates );
$path = dirname( $file ) . '/';
foreach ( self::$classes[ $file ] as $class_name ) {
if ( class_exists( $class_name ) ) {
continue;
}
$fpath = $path . substr( $class_name, 3 ) . '.php';
if ( file_exists( $fpath ) ) {
include $fpath;
self::$loaded[] = $fpath;
}
}
if ( $do_callbacks ) {
foreach ( self::$callbacks as $callback ) {
call_user_func( $callback );
}
}
}
static function get_info() {
arsort( self::$candidates );
return array( self::$loaded, self::$candidates );
}
}
endif;
if ( ! function_exists( 'scb_init' ) ) :
function scb_init( $callback = '' ) {
scbLoad4::init( $callback );
}
endif;
| {
"content_hash": "982b677dd0c66007e9d3e02f76a9d507",
"timestamp": "",
"source": "github",
"line_count": 105,
"max_line_length": 102,
"avg_line_length": 21.666666666666668,
"alnum_prop": 0.592967032967033,
"repo_name": "DietBet/Dietbet",
"id": "df20f54231335ecff7de6536741f56b50d6a1c4d",
"size": "2275",
"binary": false,
"copies": "86",
"ref": "refs/heads/master",
"path": "wp-content/plugins/wp-pagenavi/scb/load.php",
"mode": "33261",
"license": "apache-2.0",
"language": [
{
"name": "ActionScript",
"bytes": "2500"
},
{
"name": "ApacheConf",
"bytes": "6903"
},
{
"name": "CSS",
"bytes": "5859607"
},
{
"name": "HTML",
"bytes": "163168"
},
{
"name": "JavaScript",
"bytes": "6108663"
},
{
"name": "PHP",
"bytes": "36290514"
}
],
"symlink_target": ""
} |
ACCEPTED
#### According to
Index Fungorum
#### Published in
null
#### Original name
Lecidea xanthococca subsp. xanthococca Sommerf.
### Remarks
null | {
"content_hash": "928a1dc08f6102be8176f5e5e32be980",
"timestamp": "",
"source": "github",
"line_count": 13,
"max_line_length": 47,
"avg_line_length": 11.692307692307692,
"alnum_prop": 0.7302631578947368,
"repo_name": "mdoering/backbone",
"id": "3649a28a01201e1f8c6752af356b644562aefe03",
"size": "226",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "life/Fungi/Ascomycota/Lecanoromycetes/Lecanorales/Lecanoraceae/Pycnora/Pycnora xanthococca/Lecidea xanthococca xanthococca/README.md",
"mode": "33188",
"license": "apache-2.0",
"language": [],
"symlink_target": ""
} |
<sup><sup>*Release notes were automatically generated by [Shipkit](http://shipkit.org/)*</sup></sup>
#### 0.16.22
- 2019-03-24 - [4 commits](https://github.com/mockito/shipkit-example/compare/v0.16.21...v0.16.22) by [Marcin Stachniuk](https://github.com/mstachniuk) (2), [shipkit.org automated bot](https://github.com/shipkit-org) (2) - published to [](https://bintray.com/shipkit/examples/basic/0.16.22)
- Simplify implementation to trigger shipping Javadoc [(#215)](https://github.com/mockito/shipkit-example/pull/215)
- Version of shipkit upgraded to 2.2.2 [(#214)](https://github.com/mockito/shipkit-example/pull/214)
#### 0.16.21
- 2019-03-17 - [14 commits](https://github.com/mockito/shipkit-example/compare/v0.16.20...v0.16.21) by [shipkit.org automated bot](https://github.com/shipkit-org) (10), [Marcin Stachniuk](https://github.com/mstachniuk) (4) - published to [](https://bintray.com/shipkit/examples/basic/0.16.21)
- Trigger publication, to release Javadocs [(#213)](https://github.com/mockito/shipkit-example/pull/213)
- Enable Shipping Javadoc, bump Gradle version to 4.0 [(#212)](https://github.com/mockito/shipkit-example/pull/212)
- Version of shipkit upgraded to 2.2.0 [(#211)](https://github.com/mockito/shipkit-example/pull/211)
- Version of shipkit upgraded to 2.1.8 [(#210)](https://github.com/mockito/shipkit-example/pull/210)
- Version of shipkit upgraded to 2.1.7 [(#209)](https://github.com/mockito/shipkit-example/pull/209)
- Version of shipkit upgraded to 2.1.6 [(#208)](https://github.com/mockito/shipkit-example/pull/208)
- Version of shipkit upgraded to 2.1.5 [(#207)](https://github.com/mockito/shipkit-example/pull/207)
#### 0.16.20
- 2019-02-23 - [2 commits](https://github.com/mockito/shipkit-example/compare/v0.16.19...v0.16.20) by [Marcin Stachniuk](https://github.com/mstachniuk) - published to [](https://bintray.com/shipkit/examples/basic/0.16.20)
- Add comment in SomeApi, bump gradle to 3.5 [(#206)](https://github.com/mockito/shipkit-example/pull/206)
#### 0.16.19
- 2019-02-23 - [2 commits](https://github.com/mockito/shipkit-example/compare/v0.16.18...v0.16.19) by [shipkit.org automated bot](https://github.com/shipkit-org) - published to [](https://bintray.com/shipkit/examples/basic/0.16.19)
- Version of shipkit upgraded to 2.1.3 [(#205)](https://github.com/mockito/shipkit-example/pull/205)
#### 0.16.18
- 2019-02-20 - [10 commits](https://github.com/mockito/shipkit-example/compare/v0.16.15...v0.16.18) by [Marcin Stachniuk](https://github.com/mstachniuk) (4), [shipkit.org automated bot](https://github.com/shipkit-org) (4), [Erhard Pointl](https://github.com/epeee) (2) - published to [](https://bintray.com/shipkit/examples/basic/0.16.18)
- Add comment to the someLibraryMethod [(#204)](https://github.com/mockito/shipkit-example/pull/204)
- Version of shipkit upgraded to 2.1.0 [(#203)](https://github.com/mockito/shipkit-example/pull/203)
- Version of shipkit upgraded to 2.0.32 [(#202)](https://github.com/mockito/shipkit-example/pull/202)
- Add all Shipkit configuration options with comments and defaults [(#201)](https://github.com/mockito/shipkit-example/pull/201)
- Increase version [(#200)](https://github.com/mockito/shipkit-example/pull/200)
- increase version manually [(#199)](https://github.com/mockito/shipkit-example/pull/199)
#### 0.16.15
- 2018-09-08 - [18 commits](https://github.com/mockito/shipkit-example/compare/v0.16.14...v0.16.15) by [shipkit.org automated bot](https://github.com/shipkit-org) (16), [Marcin Stachniuk](https://github.com/mstachniuk) (2) - published to [](https://bintray.com/shipkit/examples/basic/0.16.15)
- Dummy change in code to perform release [(#197)](https://github.com/mockito/shipkit-example/pull/197)
- Version of shipkit upgraded to 2.0.31 [(#196)](https://github.com/mockito/shipkit-example/pull/196)
- Version of shipkit upgraded to 2.0.30 [(#195)](https://github.com/mockito/shipkit-example/pull/195)
- Version of shipkit upgraded to 2.0.29 [(#194)](https://github.com/mockito/shipkit-example/pull/194)
- Version of shipkit upgraded to 2.0.28 [(#193)](https://github.com/mockito/shipkit-example/pull/193)
- Version of shipkit upgraded to 2.0.27 [(#192)](https://github.com/mockito/shipkit-example/pull/192)
- Version of shipkit upgraded to 2.0.26 [(#191)](https://github.com/mockito/shipkit-example/pull/191)
- Version of shipkit upgraded to 2.0.25 [(#190)](https://github.com/mockito/shipkit-example/pull/190)
- Version of shipkit upgraded to 2.0.24 [(#189)](https://github.com/mockito/shipkit-example/pull/189)
#### 0.16.14
- 2018-04-26 - [17 commits](https://github.com/mockito/shipkit-example/compare/v0.16.13...v0.16.14) by [shipkit.org automated bot](https://github.com/shipkit-org) (15), [Erhard Pointl](https://github.com/epeee) (1), [Wojtek Wilk](https://github.com/wwilk) (1) - published to [](https://bintray.com/shipkit/examples/basic/0.16.14)
- minor change to trigger release [(#188)](https://github.com/mockito/shipkit-example/pull/188)
- Version of shipkit upgraded to 2.0.23 [(#187)](https://github.com/mockito/shipkit-example/pull/187)
- Version of shipkit upgraded to 2.0.20 [(#186)](https://github.com/mockito/shipkit-example/pull/186)
- Version of shipkit upgraded to 2.0.19 [(#185)](https://github.com/mockito/shipkit-example/pull/185)
- Version of shipkit upgraded to 2.0.18 [(#184)](https://github.com/mockito/shipkit-example/pull/184)
- Version of shipkit upgraded to 2.0.17 [(#183)](https://github.com/mockito/shipkit-example/pull/183)
- Version of shipkit upgraded to 2.0.16 [(#182)](https://github.com/mockito/shipkit-example/pull/182)
- Version of shipkit upgraded to 2.0.15 [(#181)](https://github.com/mockito/shipkit-example/pull/181)
- Version of shipkit upgraded to 2.0.14 [(#180)](https://github.com/mockito/shipkit-example/pull/180)
- Version of shipkit upgraded to 2.0.13 [(#179)](https://github.com/mockito/shipkit-example/pull/179)
#### 0.16.13
- 2018-03-25 - [36 commits](https://github.com/mockito/shipkit-example/compare/v0.16.12...v0.16.13) by 4 authors - published to [](https://bintray.com/shipkit/examples/basic/0.16.13)
- Commits: [shipkit.org automated bot](https://github.com/shipkit-org) (22), [Erhard Pointl](https://github.com/epeee) (12), [Szczepan Faber](https://github.com/szczepiq) (1), [Wojtek Wilk](https://github.com/wwilk) (1)
- minor change to trigger release [(#178)](https://github.com/mockito/shipkit-example/pull/178)
- Version of shipkit upgraded to 2.0.12 [(#177)](https://github.com/mockito/shipkit-example/pull/177)
- Version of shipkit upgraded to 2.0.10 [(#176)](https://github.com/mockito/shipkit-example/pull/176)
- Version of shipkit upgraded to 2.0.9 [(#175)](https://github.com/mockito/shipkit-example/pull/175)
- Version of shipkit upgraded to 2.0.8 [(#174)](https://github.com/mockito/shipkit-example/pull/174)
- Version of shipkit upgraded to 2.0.7 [(#173)](https://github.com/mockito/shipkit-example/pull/173)
- Version of shipkit upgraded to 2.0.6 [(#172)](https://github.com/mockito/shipkit-example/pull/172)
- update shipkit to v2.0.4 [(#171)](https://github.com/mockito/shipkit-example/pull/171)
- update shipkit to v2.0.2 [(#170)](https://github.com/mockito/shipkit-example/pull/170)
- Version of shipkit upgraded to 2.0.1 [(#169)](https://github.com/mockito/shipkit-example/pull/169)
- Version of shipkit upgraded to 2.0.0 [(#168)](https://github.com/mockito/shipkit-example/pull/168)
- Version of shipkit upgraded to 1.0.6 [(#167)](https://github.com/mockito/shipkit-example/pull/167)
- Version of shipkit upgraded to 1.0.4 [(#166)](https://github.com/mockito/shipkit-example/pull/166)
- Version of shipkit upgraded to 0.9.149 [(#160)](https://github.com/mockito/shipkit-example/pull/160)
- Version of shipkit upgraded to 0.9.147 [(#157)](https://github.com/mockito/shipkit-example/pull/157)
- Version of shipkit upgraded to 0.9.146 [(#156)](https://github.com/mockito/shipkit-example/pull/156)
- Version of shipkit upgraded to 0.9.144 [(#154)](https://github.com/mockito/shipkit-example/pull/154)
**0.16.12 (2017-11-16)** - [4 commits](https://github.com/mockito/shipkit-example/compare/v0.16.11...v0.16.12) by [Erhard Pointl](https://github.com/epeee) (3), [shipkit-org](https://github.com/shipkit-org) (1) - published to [](https://bintray.com/shipkit/examples/basic0.16.12)
- minor change in test class [(#153)](https://github.com/mockito/shipkit-example/pull/153)
- Version of shipkit upgraded to 0.9.143 [(#152)](https://github.com/mockito/shipkit-example/pull/152)
**0.16.11 (2017-11-03)** - [2 commits](https://github.com/mockito/shipkit-example/compare/v0.16.10...v0.16.11) by [shipkit-org](https://github.com/shipkit-org) (1), [Wojtek Wilk](http://github.com/wwilk) (1) - published to [](https://bintray.com/shipkit/examples/basic0.16.11)
- Version of shipkit upgraded to 0.9.133 [(#144)](https://github.com/mockito/shipkit-example/pull/144)
**0.16.10 (2017-11-01)** - [2 commits](https://github.com/mockito/shipkit-example/compare/v0.16.9...v0.16.10) by [shipkit-org](https://github.com/shipkit-org) (1), [Wojtek Wilk](http://github.com/wwilk) (1) - published to [](https://bintray.com/shipkit/examples/basic0.16.10)
- Version of shipkit upgraded to 0.9.130 [(#141)](https://github.com/mockito/shipkit-example/pull/141)
**0.16.9 (2017-10-29)** - [2 commits](https://github.com/mockito/shipkit-example/compare/v0.16.8...v0.16.9) by [shipkit-org](https://github.com/shipkit-org) (1), [Wojtek Wilk](http://github.com/wwilk) (1) - published to [](https://bintray.com/shipkit/examples/basic0.16.9)
- Version of shipkit upgraded to 0.9.127 [(#137)](https://github.com/mockito/shipkit-example/pull/137)
**0.16.8 (2017-10-28)** - [44 commits](https://github.com/mockito/shipkit-example/compare/v0.16.7...v0.16.8) by 6 authors - published to [](https://bintray.com/shipkit/examples/basic/0.16.8)
- Commits: [Szczepan Faber](http://github.com/szczepiq) (13), Travis CI User (13), [Erhard Pointl](https://github.com/epeee) (5), [Wojtek Wilk](http://github.com/wwilk) (5), Mockito Release Tools (4), [shipkit-org](https://github.com/shipkit-org) (4)
- Version of shipkit upgraded to 0.9.122 [(#132)](https://github.com/mockito/shipkit-example/pull/132)
- Version of shipkit upgraded to 0.9.117 [(#127)](https://github.com/mockito/shipkit-example/pull/127)
- Version of shipkit upgraded to 0.9.110 [(#121)](https://github.com/mockito/shipkit-example/pull/121)
- Version of shipkit upgraded to 0.9.106 [(#117)](https://github.com/mockito/shipkit-example/pull/117)
- Version of shipkit upgraded to 0.9.97 [(#108)](https://github.com/mockito/shipkit-example/pull/108)
- Version of shipkit upgraded to 0.9.89 [(#100)](https://github.com/mockito/shipkit-example/pull/100)
- Version of shipkit upgraded to 0.9.85 [(#96)](https://github.com/mockito/shipkit-example/pull/96)
- Bumped Shipkit and updated plugin id [(#94)](https://github.com/mockito/shipkit-example/pull/94)
- Version of shipkit upgraded to 0.9.63 [(#93)](https://github.com/mockito/shipkit-example/pull/93)
- Version of shipkit upgraded to 0.9.62 [(#92)](https://github.com/mockito/shipkit-example/pull/92)
- Version of shipkit upgraded to 0.9.58 [(#91)](https://github.com/mockito/shipkit-example/pull/91)
- Version of shipkit upgraded to 0.9.56 [(#89)](https://github.com/mockito/shipkit-example/pull/89)
- Version of shipkit upgraded to 0.9.55 [(#88)](https://github.com/mockito/shipkit-example/pull/88)
- Version of shipkit upgraded to 0.9.53 [(#86)](https://github.com/mockito/shipkit-example/pull/86)
- Version of shipkit upgraded to 0.9.52 [(#85)](https://github.com/mockito/shipkit-example/pull/85)
- Version of shipkit upgraded to 0.9.51 [(#84)](https://github.com/mockito/shipkit-example/pull/84)
- Version of shipkit upgraded to 0.9.49 [(#82)](https://github.com/mockito/shipkit-example/pull/82)
- Version of shipkit upgraded to 0.9.46 [(#79)](https://github.com/mockito/shipkit-example/pull/79)
- Bumped Shipkit + tidy-up [(#76)](https://github.com/mockito/shipkit-example/pull/76)
- Shipkit version bumped and upgrade-dependency plugin applied [(#75)](https://github.com/mockito/shipkit-example/pull/75)
**0.16.7 (2017-08-08)** - [15 commits](https://github.com/mockito/shipkit-example/compare/v0.16.5...v0.16.7) by [Wojtek Wilk](http://github.com/wwilk) (7), [Erhard Pointl](https://github.com/epeee) (4), Mockito Release Tools (4) - published to [](https://bintray.com/shipkit/examples/basic/0.16.7)
- make use of latest shipkit version [(#73)](https://github.com/mockito/shipkit-example/pull/73)
- VersionUpgradeConsumerPlugin applied [(#71)](https://github.com/mockito/shipkit-example/pull/71)
- publications-comparator plugin renamed to compare-publications [(#63)](https://github.com/mockito/shipkit-example/pull/63)
- Bumped shipkit & some tidy-up [(#62)](https://github.com/mockito/shipkit-example/pull/62)
**0.16.6 (2017-07-03)** - no code changes (no commits) - published to [](https://bintray.com/shipkit/examples/basic/0.16.6)
**0.16.5 (2017-07-02)** - [22 commits](https://github.com/mockito/shipkit-example/compare/v0.16.3...v0.16.5) by 6 authors - published to [](https://bintray.com/shipkit/examples/basic/0.16.5)
- Commits: Mockito Release Tools (10), [Maciej Kuster](https://github.com/NagRock) (4), [Marcin Stachniuk](http://github.com/mstachniuk) (4), [Erhard Pointl](https://github.com/epeee) (2), [Szczepan Faber](http://github.com/szczepiq) (1), [Wojtek Wilk](http://github.com/wwilk) (1)
- Change implementation to make a new release [(#59)](https://github.com/mockito/shipkit-example/pull/59)
- Bumped shipkit [(#58)](https://github.com/mockito/shipkit-example/pull/58)
- make use of latest shipkit version (v0.8.90) [(#56)](https://github.com/mockito/shipkit-example/pull/56)
- added default for releaseNotes.ignoreCommitsContaining [(#43)](https://github.com/mockito/shipkit-example/pull/43)
**0.16.4 (2017-07-02)** - [21 commits](https://github.com/mockito/shipkit-example/compare/v0.16.3...v0.16.4) by 5 authors - published to [](https://bintray.com/shipkit/examples/basic/0.16.4)
- Commits: Mockito Release Tools (10), [Maciej Kuster](https://github.com/NagRock) (4), [Marcin Stachniuk](http://github.com/mstachniuk) (4), [Erhard Pointl](https://github.com/epeee) (2), [Szczepan Faber](http://github.com/szczepiq) (1)
- Change implementation to make a new release [(#59)](https://github.com/mockito/shipkit-example/pull/59)
- Bumped shipkit [(#58)](https://github.com/mockito/shipkit-example/pull/58)
- make use of latest shipkit version (v0.8.90) [(#56)](https://github.com/mockito/shipkit-example/pull/56)
- added default for releaseNotes.ignoreCommitsContaining [(#43)](https://github.com/mockito/shipkit-example/pull/43)
**0.16.3 (2017-06-08)** - no code changes (no commits) - published to [](https://bintray.com/shipkit/examples/basic/0.16.3)
**0.16.2 (2017-06-08)** - no code changes (no commits) - published to [](https://bintray.com/shipkit/examples/basic/0.16.2)
**0.16.1 (2017-06-08)** - [36 commits](https://github.com/mockito/shipkit-example/compare/v0.15.2...v0.16.1) by 6 authors - published to [](https://bintray.com/shipkit/examples/basic/0.16.1)
- Commits: [Marcin Stachniuk](http://github.com/mstachniuk) (9), Mockito Release Tools (8), [Szczepan Faber](http://github.com/szczepiq) (7), [Wojtek Wilk](http://github.com/wwilk) (5), [Erhard Pointl](https://github.com/epeee) (4), [mkuster](https://github.com/NagRock) (3)
- Continuation of renaming from mockito-release-tools into shipkit [(#52)](https://github.com/mockito/shipkit-example/pull/52)
- Version bumped [(#51)](https://github.com/mockito/shipkit-example/pull/51)
- Downgrade previous version to 0.15.2 [(#50)](https://github.com/mockito/shipkit-example/pull/50)
- Renamed MockitoReleaseTools dependency to Shipkit [(#49)](https://github.com/mockito/shipkit-example/pull/49)
- Clean up shipkit Gradle plugin names [(#44)](https://github.com/mockito/shipkit-example/pull/44)
- Gradle extension name changed from 'releasing' to 'shipkit' [(#42)](https://github.com/mockito/shipkit-example/pull/42)
- Bumped version of tools [(#37)](https://github.com/mockito/shipkit-example/pull/37)
- Bump release tools version to 0.8.32 [(#35)](https://github.com/mockito/shipkit-example/pull/35)
- Change mockito-release-tools for easier bump by e2e tests [(#33)](https://github.com/mockito/shipkit-example/pull/33)
- Bumped release tools to 0.8.4 - concise release notes [(#32)](https://github.com/mockito/shipkit-example/pull/32)
- Bumped version of tools [(#30)](https://github.com/mockito/shipkit-example/pull/30)
- Picked up new version of tools [(#29)](https://github.com/mockito/shipkit-example/pull/29)
- make use of mockito-release-tools v0.7.5 and configure commit message [(#28)](https://github.com/mockito/shipkit-example/pull/28)
**0.16.0 (2017-06-06)** - [33 commits](https://github.com/mockito/mockito-release-tools-example/compare/v0.15.2...v0.16.0) by 6 authors - published to [](https://bintray.com/shipkit/examples/basic/0.16.0)
- Commits: [Marcin Stachniuk](http://github.com/mstachniuk) (9), Mockito Release Tools (7), [Szczepan Faber](http://github.com/szczepiq) (7), [Wojtek Wilk](http://github.com/wwilk) (5), [Erhard Pointl](https://github.com/epeee) (4), mkuster (1)
- Version bumped [(#51)](https://github.com/mockito/mockito-release-tools-example/pull/51)
- Downgrade previous version to 0.15.2 [(#50)](https://github.com/mockito/mockito-release-tools-example/pull/50)
- Renamed MockitoReleaseTools dependency to Shipkit [(#49)](https://github.com/mockito/mockito-release-tools-example/pull/49)
- Clean up shipkit Gradle plugin names [(#44)](https://github.com/mockito/mockito-release-tools-example/pull/44)
- Gradle extension name changed from 'releasing' to 'shipkit' [(#42)](https://github.com/mockito/mockito-release-tools-example/pull/42)
- Bumped version of tools [(#37)](https://github.com/mockito/mockito-release-tools-example/pull/37)
- Bump release tools version to 0.8.32 [(#35)](https://github.com/mockito/mockito-release-tools-example/pull/35)
- Change mockito-release-tools for easier bump by e2e tests [(#33)](https://github.com/mockito/mockito-release-tools-example/pull/33)
- Bumped release tools to 0.8.4 - concise release notes [(#32)](https://github.com/mockito/mockito-release-tools-example/pull/32)
- Bumped version of tools [(#30)](https://github.com/mockito/mockito-release-tools-example/pull/30)
- Picked up new version of tools [(#29)](https://github.com/mockito/mockito-release-tools-example/pull/29)
- make use of mockito-release-tools v0.7.5 and configure commit message [(#28)](https://github.com/mockito/mockito-release-tools-example/pull/28)
**0.15.11 (2017-06-06)** - [32 commits](https://github.com/mockito/mockito-release-tools-example/compare/v0.15.2...v0.15.11) by 6 authors - published to [](https://bintray.com/shipkit/examples/basic/0.15.11)
- Commits: [Marcin Stachniuk](http://github.com/mstachniuk) (9), Mockito Release Tools (7), [Szczepan Faber](http://github.com/szczepiq) (7), [Erhard Pointl](https://github.com/epeee) (4), [Wojtek Wilk](http://github.com/wwilk) (4), mkuster (1)
- Downgrade previous version to 0.15.2 [(#50)](https://github.com/mockito/mockito-release-tools-example/pull/50)
- Renamed MockitoReleaseTools dependency to Shipkit [(#49)](https://github.com/mockito/mockito-release-tools-example/pull/49)
- Clean up shipkit Gradle plugin names [(#44)](https://github.com/mockito/mockito-release-tools-example/pull/44)
- Gradle extension name changed from 'releasing' to 'shipkit' [(#42)](https://github.com/mockito/mockito-release-tools-example/pull/42)
- Bumped version of tools [(#37)](https://github.com/mockito/mockito-release-tools-example/pull/37)
- Bump release tools version to 0.8.32 [(#35)](https://github.com/mockito/mockito-release-tools-example/pull/35)
- Change mockito-release-tools for easier bump by e2e tests [(#33)](https://github.com/mockito/mockito-release-tools-example/pull/33)
- Bumped release tools to 0.8.4 - concise release notes [(#32)](https://github.com/mockito/mockito-release-tools-example/pull/32)
- Bumped version of tools [(#30)](https://github.com/mockito/mockito-release-tools-example/pull/30)
- Picked up new version of tools [(#29)](https://github.com/mockito/mockito-release-tools-example/pull/29)
- make use of mockito-release-tools v0.7.5 and configure commit message [(#28)](https://github.com/mockito/mockito-release-tools-example/pull/28)
**0.15.10 (2017-06-02)** - no code changes (no commits) - published to [](https://bintray.com/shipkit/examples/basic/0.15.10)
**0.15.9 (2017-06-02)** - no code changes (no commits) - published to [](https://bintray.com/shipkit/examples/basic/0.15.9)
**0.15.8 (2017-06-02)** - no code changes (no commits) - published to [](https://bintray.com/shipkit/examples/basic/0.15.8)
**0.15.7 (2017-06-01)** - [26 commits](https://github.com/mockito/mockito-release-tools-example/compare/v0.15.2...v0.15.7) by 6 authors - published to [](https://bintray.com/shipkit/examples/basic/0.15.7)
- Commits: [Marcin Stachniuk](http://github.com/mstachniuk) (7), Mockito Release Tools (7), [Szczepan Faber](http://github.com/szczepiq) (7), [Wojtek Wilk](http://github.com/wwilk) (3), epeee (1), mkuster (1)
- Gradle extension name changed from 'releasing' to 'shipkit' [(#42)](https://github.com/mockito/mockito-release-tools-example/pull/42)
- Bumped version of tools [(#37)](https://github.com/mockito/mockito-release-tools-example/pull/37)
- Bump release tools version to 0.8.32 [(#35)](https://github.com/mockito/mockito-release-tools-example/pull/35)
- Change mockito-release-tools for easier bump by e2e tests [(#33)](https://github.com/mockito/mockito-release-tools-example/pull/33)
- Bumped release tools to 0.8.4 - concise release notes [(#32)](https://github.com/mockito/mockito-release-tools-example/pull/32)
- Bumped version of tools [(#30)](https://github.com/mockito/mockito-release-tools-example/pull/30)
- Picked up new version of tools [(#29)](https://github.com/mockito/mockito-release-tools-example/pull/29)
- make use of mockito-release-tools v0.7.5 and configure commit message [(#28)](https://github.com/mockito/mockito-release-tools-example/pull/28)
**0.15.6 (2017-06-01)** - [25 commits](https://github.com/mockito/mockito-release-tools-example/compare/v0.15.2...v0.15.6) by 6 authors - published to [](https://bintray.com/shipkit/examples/basic/0.15.6)
- Commits: [Marcin Stachniuk](http://github.com/mstachniuk) (7), [Szczepan Faber](http://github.com/szczepiq) (7), Mockito Release Tools (6), [Wojtek Wilk](http://github.com/wwilk) (3), epeee (1), mkuster (1)
- Gradle extension name changed from 'releasing' to 'shipkit' [(#42)](https://github.com/mockito/mockito-release-tools-example/pull/42)
- Bumped version of tools [(#37)](https://github.com/mockito/mockito-release-tools-example/pull/37)
- Bump release tools version to 0.8.32 [(#35)](https://github.com/mockito/mockito-release-tools-example/pull/35)
- Change mockito-release-tools for easier bump by e2e tests [(#33)](https://github.com/mockito/mockito-release-tools-example/pull/33)
- Bumped release tools to 0.8.4 - concise release notes [(#32)](https://github.com/mockito/mockito-release-tools-example/pull/32)
- Bumped version of tools [(#30)](https://github.com/mockito/mockito-release-tools-example/pull/30)
- Picked up new version of tools [(#29)](https://github.com/mockito/mockito-release-tools-example/pull/29)
- make use of mockito-release-tools v0.7.5 and configure commit message [(#28)](https://github.com/mockito/mockito-release-tools-example/pull/28)
**0.15.5** - no code changes (no commits) - *2017-05-25* - published to [examples/basic](https://bintray.com/shipkit/examples/basic)
**0.15.4** - no code changes (no commits) - *2017-05-25* - published to [examples/basic](https://bintray.com/shipkit/examples/basic)
**0.15.3** - [20 commits](https://github.com/mockito/mockito-release-tools-example/compare/v0.15.2...v0.15.3) by Mockito Release Tools - *2017-05-25* - published to [examples/basic](https://bintray.com/shipkit/examples/basic)
- Bumped version of tools [(#37)](https://github.com/mockito/mockito-release-tools-example/pull/37)
- Bump release tools version to 0.8.32 [(#35)](https://github.com/mockito/mockito-release-tools-example/pull/35)
- Change mockito-release-tools for easier bump by e2e tests [(#33)](https://github.com/mockito/mockito-release-tools-example/pull/33)
- Bumped release tools to 0.8.4 - concise release notes [(#32)](https://github.com/mockito/mockito-release-tools-example/pull/32)
- Bumped version of tools [(#30)](https://github.com/mockito/mockito-release-tools-example/pull/30)
- Picked up new version of tools [(#29)](https://github.com/mockito/mockito-release-tools-example/pull/29)
- make use of mockito-release-tools v0.7.5 and configure commit message [(#28)](https://github.com/mockito/mockito-release-tools-example/pull/28)
### 0.15.2 (2017-04-29)
* Authors: 0
* Commits: 0
* No notable improvements. See the commits for detailed changes.
### 0.15.1 (2017-04-23)
* Authors: 1
* Commits: 9
* 9: [Szczepan Faber](https://github.com/szczepiq)
* Improvements: 1
* Pulled new version of tools [(#27)](https://github.com/mockito/mockito-release-tools-example/pull/27)
### 0.15.0 (2017-04-11)
* Authors: 1
* Commits: 2
* 2: [Szczepan Faber](https://github.com/szczepiq)
* Improvements: 1
* Fixed the release [(#26)](https://github.com/mockito/mockito-release-tools-example/pull/26)
### 0.14.0 (2017-04-11)
* Authors: 1
* Commits: 3
* 3: [Szczepan Faber](https://github.com/szczepiq)
* Improvements: 1
* Publishing notable versions to separate Bintray package instead of Bintray repo [(#25)](https://github.com/mockito/mockito-release-tools-example/pull/25)
### 0.13.5 (2017-04-03)
* Authors: 1
* Commits: 16
* 16: [Szczepan Faber](https://github.com/szczepiq)
* Improvements: 4
* Picked up new version of tools [(#24)](https://github.com/mockito/mockito-release-tools-example/pull/24)
* Picked up latest release tools [(#23)](https://github.com/mockito/mockito-release-tools-example/pull/23)
* Notable release support [(#22)](https://github.com/mockito/mockito-release-tools-example/pull/22)
* Reduced the complexity [(#21)](https://github.com/mockito/mockito-release-tools-example/pull/21)
### 0.2.0 (2017-03-26)
* Authors: 0
* Commits: 0
* No notable improvements. See the commits for detailed changes.
### 0.11.5 (2017-03-25)
* Authors: 0
* Commits: 0
* No notable improvements. See the commits for detailed changes.
### 0.11.4 (2017-03-25)
* Authors: 0
* Commits: 0
* No notable improvements. See the commits for detailed changes.
### 0.11.3 (2017-03-25)
* Authors: 1
* Commits: 1
* 1: [Szczepan Faber](https://github.com/szczepiq)
* No notable improvements. See the commits for detailed changes.
### 0.11.2 (2017-03-25)
* Authors: 1
* Commits: 2
* 2: [Szczepan Faber](https://github.com/szczepiq)
* Improvements: 1
* Refactoring: Fixes env var to TERM for readability purpose on travis log when building with gradle [(#19)](https://github.com/mockito/mockito/pull/19)
### 0.11.1 (2017-03-23)
* Authors: 1
* Commits: 6
* 6: [Szczepan Faber](https://github.com/szczepiq)
* Improvements: 1
* Enabled continuous integration with Travis CI and coverage tracking with coveralls [(#18)](https://github.com/mockito/mockito/pull/18)
### 0.11.0 (2017-03-22)
* Authors: 1
* Commits: 5
* 5: [Szczepan Faber](https://github.com/szczepiq)
* Improvements: 1
* Fix for problem with classloaders on Eclipse plugins tests. [(#17)](https://github.com/mockito/mockito/pull/17)
### 0.10.0 (2017-03-20)
* Authors: 1
* Commits: 3
* 3: [Szczepan Faber](https://github.com/szczepiq)
* Improvements: 1
* Adds an after() method, which acts like timeout() but does not immediately return if the test passes [(#16)](https://github.com/mockito/mockito/pull/16)
### 0.9.1 (2017-03-18)
* Authors: 1
* Commits: 5
* 5: [Szczepan Faber](https://github.com/szczepiq)
* Improvements: 1
* Verification with timout measures time more more accurately [(#15)](https://github.com/mockito/mockito/pull/15)
### 0.9.0 (2017-03-16)
* Authors: 1
* Commits: 12
* 12: [Szczepan Faber](https://github.com/szczepiq)
* Improvements: 3
* Deprecated timeout().never(), in line with timeout().atMost() [(#14)](https://github.com/mockito/mockito/pull/14)
* Refactoring: Remove old Hg artifacts [(#13)](https://github.com/mockito/mockito/pull/13)
* Upgrade to gradle 1.8 [(#12)](https://github.com/mockito/mockito/pull/12)
### 0.7.1 (2017-03-15)
* Authors: 1
* Commits: 2
* 2: Szczepan Faber
* Improvements: 1
* Improved logging and made the build safer [(#11)](https://github.com/mockito/mockito-release-tools-example/pull/11)
### 0.7.0 (2017-03-15)
* Authors: 1
* Commits: 35
* 35: Szczepan Faber
* Improvements: 6
* Fixed problem with git user in Travis CI [(#10)](https://github.com/mockito/mockito-release-tools-example/pull/10)
* Fixed various Travis CI configuration issues [(#9)](https://github.com/mockito/mockito-release-tools-example/pull/9)
* Extracted release automation to script plugin [(#8)](https://github.com/mockito/mockito-release-tools-example/pull/8)
* Set up release automation [(#7)](https://github.com/mockito/mockito-release-tools-example/pull/7)
* Example project publishes to Bintray and has version management [(#6)](https://github.com/mockito/mockito-release-tools-example/pull/6)
* Configured release notes repository [(#4)](https://github.com/mockito/mockito-release-tools-example/pull/4)
### 0.3.0 (2017-03-12)
* Authors: 1
* Commits: 2
* 2: Szczepan Faber
* No notable improvements. See the commits for detailed changes.
### 0.2.2 (2017-03-12)
* Authors: 0
* Commits: 0
* No notable improvements. See the commits for detailed changes.
### 0.2.1 (2017-03-12)
* Authors: 1
* Commits: 22
* 22: Szczepan Faber
* Improvements: 2
* Automated release notes generation [(#3)](https://github.com/mockito/mockito-release-tools-example/pull/3)
* Initial check-in of sample set of libraries for testing releases [(#1)](https://github.com/mockito/mockito-release-tools-example/pull/1)
### 0.0.1 initial
To get started with | {
"content_hash": "9fa1541362a6cc01ab855f013dd8390f",
"timestamp": "",
"source": "github",
"line_count": 390,
"max_line_length": 405,
"avg_line_length": 82.13846153846154,
"alnum_prop": 0.7206717862271337,
"repo_name": "mockito/shipkit-example",
"id": "edd384ed1ff45c9e5243b25fa40d30a126061ec8",
"size": "32034",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "docs/release-notes.md",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "Java",
"bytes": "765"
}
],
"symlink_target": ""
} |
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace CapsLockIndicatorV3
{
public partial class PaddingInputDialog : DarkModeForm
{
private Color controlBackColor;
protected override void WndProc(ref Message message)
{
if (message.Msg == 0x0084) // WM_NCHITTEST
message.Result = (IntPtr)1;
else base.WndProc(ref message);
}
public Padding Value => new Padding(
(int)numericUpDownLeft.Value,
(int)numericUpDownTop.Value,
(int)numericUpDownRight.Value,
(int)numericUpDownBottom.Value
);
public PaddingInputDialog(Padding defaultValue)
{
InitializeComponent();
numericUpDownLeft.Maximum =
numericUpDownTop.Maximum =
numericUpDownRight.Maximum =
numericUpDownBottom.Maximum = int.MaxValue;
numericUpDownLeft.Value = defaultValue.Left;
numericUpDownTop.Value = defaultValue.Top;
numericUpDownRight.Value = defaultValue.Right;
numericUpDownBottom.Value = defaultValue.Bottom;
HandleCreated += (sender, e) =>
{
DarkModeChanged += TextInputDialog_DarkModeChanged;
DarkModeProvider.RegisterForm(this);
};
}
private void TextInputDialog_DarkModeChanged(object sender, EventArgs e)
{
var dark = DarkModeProvider.IsDark;
Native.UseImmersiveDarkModeColors(Handle, dark);
ForeColor =
numericUpDownLeft.ForeColor =
numericUpDownTop.ForeColor =
numericUpDownRight.ForeColor =
numericUpDownBottom.ForeColor =
dark ? Color.White : SystemColors.WindowText;
numericUpDownLeft.BackColor =
numericUpDownTop.BackColor =
numericUpDownRight.BackColor =
numericUpDownBottom.BackColor =
controlBackColor =
dark ? Color.FromArgb(255, 56, 56, 56) : SystemColors.Window;
numericUpDownLeft.BorderStyle =
numericUpDownTop.BorderStyle =
numericUpDownRight.BorderStyle =
numericUpDownBottom.BorderStyle =
dark ? BorderStyle.FixedSingle : BorderStyle.Fixed3D;
BackColor = dark ? Color.FromArgb(255, 32, 32, 32) : SystemColors.Window;
ControlScheduleSetDarkMode(numericUpDownLeft, dark);
ControlScheduleSetDarkMode(numericUpDownTop, dark);
ControlScheduleSetDarkMode(numericUpDownRight, dark);
ControlScheduleSetDarkMode(numericUpDownBottom, dark);
ControlScheduleSetDarkMode(okButton, dark);
}
private void button1_Click(object sender, EventArgs e)
{
DialogResult = DialogResult.OK;
Close();
}
private void centerControl_Paint(object sender, PaintEventArgs e)
{
//ControlPaint.DrawBorder3D(e.Graphics, new Rectangle(Point.Empty, centerControl.Size));
//ButtonRenderer.DrawButton(e.Graphics, new Rectangle(Point.Empty, centerControl.Size), )
//ControlPaint.DrawButton(e.Graphics, new Rectangle(Point.Empty, centerControl.Size), ButtonState.Normal);
ControlPaint.DrawBorder(e.Graphics, new Rectangle(Point.Empty, centerControl.Size), controlBackColor, ButtonBorderStyle.Outset);
}
}
}
| {
"content_hash": "4d92a448e20309a16f38cc584ff1c2fc",
"timestamp": "",
"source": "github",
"line_count": 100,
"max_line_length": 140,
"avg_line_length": 36.1,
"alnum_prop": 0.6343490304709142,
"repo_name": "jonaskohl/CapsLockIndicator",
"id": "af85517430b76e2f23083af76a78efade890ac9b",
"size": "3612",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "CapsLockIndicatorV3/PaddingInputDialog.cs",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "C#",
"bytes": "282228"
},
{
"name": "PowerShell",
"bytes": "139"
}
],
"symlink_target": ""
} |
/**
* Created by alammar on 12/8/16.
*/
var shallowNeuralNetworkGrapher = function (inputNodeCount, outputNodeCount, svgElement, analyticsCategory) {
this.inputNodeCount = inputNodeCount;
this.outputNodeCount = outputNodeCount;
this.svgElement = svgElement;
this.graphWidth = 350;
this.analyticsCategory = analyticsCategory;
this.initializeGraph();
this.drawGraph()
};
shallowNeuralNetworkGrapher.prototype.updateNodeCount = function (nodeType, newValue){
if( nodeType == "input" ) this.inputNodeCount = newValue;
else if ( nodeType == "output" ) this.outputNodeCount = newValue;
this.drawGraph();
};
shallowNeuralNetworkGrapher.prototype.initializeGraph = function () {
this.nnGraphHolder = d3.select(this.svgElement) // select the 'body' element
.append("svg") // append an SVG element to the body
.attr("width", this.graphWidth) // make the SVG element 449 pixels wide
.attr("height", 250); // make the SVG element 249 pixels high
this.neuralNetworkMargin = {top: 10, right: 10, bottom: 10, left: 10},
this.neuralNetworkWidth = +this.nnGraphHolder.attr("width") - this.neuralNetworkMargin.left - this.neuralNetworkMargin.right,
this.neuralNetworkHeight = +this.nnGraphHolder.attr("height") - this.neuralNetworkMargin.top - this.neuralNetworkMargin.bottom,
this.neuralNetworkG = this.nnGraphHolder.append("g");
// Define arrow head
// http://bl.ocks.org/tomgp/d59de83f771ca2b6f1d4
var defs = this.nnGraphHolder.append("defs");
defs.append("marker")
.attrs({
"id": "arrow",
"viewBox": "0 -5 10 10",
"refX": 5,
"refY": 0,
"markerWidth": 4,
"markerHeight": 4,
"orient": "auto"
})
.append("path")
.attr("d", "M0,-5L10,0L0,5")
.attr("class", "arrowHead");
};
shallowNeuralNetworkGrapher.prototype.drawGraph = function () {
var grapher = this;
this.nodeRadius = 15;
this.weightNodeWidthRatio = 1.7;
// Let's calculate our coordinates for all the nodes
// Let's start with the X coordiantes for each layer
var inputLayerX = this.neuralNetworkMargin.left + this.nodeRadius, // X value of input layer
biasLayerX = this.neuralNetworkWidth * 2 / 3 - 20, //
outputLayerX = this.neuralNetworkWidth - this.nodeRadius + this.neuralNetworkMargin.left,
softmaxLayerX = (outputLayerX + biasLayerX) / 2;
// Now the Y coordinates for each layer
// Input layer
this.inputLayerCoordinates = d3.range(this.inputNodeCount).map(function (i) {
var y = grapher.neuralNetworkMargin.top + (i + 1) * grapher.neuralNetworkHeight / (grapher.inputNodeCount + 1);
return {x: inputLayerX, y: y, index:i};
});
// Bias layer
this.biasLayerCoordinates = d3.range(this.outputNodeCount).map(function (i) {
var y = grapher.neuralNetworkMargin.top + (i + 1) * grapher.neuralNetworkHeight / (grapher.outputNodeCount + 1);
return {x: biasLayerX, y: y, index: i};
});
// Output layer
this.outputLayerCoordinates = d3.range(this.outputNodeCount).map(function (i) {
var y = grapher.neuralNetworkMargin.top + (i + 1) * grapher.neuralNetworkHeight / (grapher.outputNodeCount + 1);
return {x: outputLayerX, y: y, index:i};
});
// Weights
// Calculate weight node locations
var weightMarginLeft = grapher.nodeRadius +grapher.nodeRadius * grapher.weightNodeWidthRatio + 15,
weightMarginRight = weightMarginLeft,
weightRegion = biasLayerX - inputLayerX - weightMarginLeft - weightMarginRight;
// calculate weight layer x coordinate (one "layer" per output) // Scales for the axes
this.weightNodeX = d3.scaleLinear()
.domain([0, this.outputNodeCount -1])
.range([inputLayerX + weightMarginLeft, biasLayerX - weightMarginRight]);
this.weightLayerXCoordinates = d3.range(this.outputNodeCount).map(function(i){
return grapher.weightNodeX(i);
});
// Calculate the angles of the all the Input-to_Bias lines
this.lineAngles = [];
for (var j = 0; j < this.outputNodeCount; j++)
for (var i = 0; i < this.inputNodeCount; i++){
//initialize inner array
if( i == 0 ) this.lineAngles[j] = new Array(this.inputNodeCount);
var opposite = this.inputLayerCoordinates[i].y - this.outputLayerCoordinates[j].y,
adjacent = biasLayerX - inputLayerX,
angle = Math.atan(opposite/adjacent);
this.lineAngles[j][i] = angle;
}
this.weightLayerCoordinates = [];
for (var i = 0; i < this.inputNodeCount; i++)
for (var j = 0; j < this.outputNodeCount; j++) {
this.weightLayerCoordinates.push({
x: this.weightLayerXCoordinates[j],
y: this.inputLayerCoordinates[i].y -
Math.tan(this.lineAngles[j][i]) *
(this.weightLayerXCoordinates[j] - inputLayerX),
outputIndex: j,
inputIndex: i
})
}
this.inputToBiasLines = [];
// Calculate the coordiantes of the lines from input to bias
for (var i = 0; i < this.inputNodeCount; i++)
for (var j = 0; j < this.outputNodeCount; j++) {
this.inputToBiasLines.push({
x1: this.inputLayerCoordinates[i].x,
y1: this.inputLayerCoordinates[i].y,
x2: this.biasLayerCoordinates[j].x,
y2: this.biasLayerCoordinates[j].y
});
}
this.BiasToSoftmaxLines = [];
// Calculate the coordiantes of the lines from input to bias
for (var i = 0; i < this.outputNodeCount; i++)
this.BiasToSoftmaxLines.push({
x1: this.biasLayerCoordinates[i].x,
y1: this.biasLayerCoordinates[i].y,
x2: softmaxLayerX - grapher.nodeRadius,
y2: this.biasLayerCoordinates[i].y
});
this.softmaxtoOutputLines = [];
// Calculate the coordiantes of the lines from input to bias
for (var i = 0; i < this.outputNodeCount; i++)
this.softmaxtoOutputLines.push({
x1: softmaxLayerX + grapher.nodeRadius + 6,
y1: this.biasLayerCoordinates[i].y,
x2: outputLayerX - grapher.nodeRadius - 8,
y2: this.biasLayerCoordinates[i].y
});
var softmaxCoordinates = {x: softmaxLayerX, y: this.neuralNetworkMargin.top};
// Graph all the things
this.graphSoftmaxToOutputArrows(this.softmaxtoOutputLines);
this.graphBiasToSoftmaxArrows(this.BiasToSoftmaxLines);
this.graphInputToBiasLines(this.inputToBiasLines);
this.graphInputNodes(this.inputLayerCoordinates);
this.graphWeightNodes(this.weightLayerCoordinates);
this.graphOutputNodes(this.outputLayerCoordinates);
this.graphBiasNodes(this.biasLayerCoordinates);
this.graphSoftmax(softmaxCoordinates);
var biasNodeY = this.neuralNetworkMargin.top + this.neuralNetworkHeight / 2 - this.nodeRadius;
};
shallowNeuralNetworkGrapher.prototype.graphInputNodes = function (data) {
// JOIN
var inputGroups = this.neuralNetworkG.selectAll(this.svgElement + " .input-group" )
.data(data);
// EXIT old elements not present in new data.
inputGroups.exit()
.attr("class", "exit")
.remove();
// UPDATE old elements present in new data.
inputGroups.attr("class", "input-group")
.attr("transform", function(d){
return "translate(" +
(d.x)
+ ","
+ (d.y) + ")";
}.bind(this));
inputGroups.select("#input-name")
.text(function(d){
return "X" + (d.index +1)
});
// ENTER new elements present in new data.
var inputs = inputGroups.enter()
.append("g")
.attr("class", "input-group")
.attr("transform", function(d){
return "translate(" +
(d.x)
+ ","
+ (d.y) + ")";
}.bind(this));
inputs
.append("circle")
.attr("class", "input-node")
.attr("r", this.nodeRadius)
.attr("cx", 0)
.attr("cy", 0);
inputs.append("text")
.attr("id", "input-name")
.attr("class", "grapher-node-text")
.attr("text-anchor", "middle")
.attr("x", 0)
.attr("y", 5)
.text(function(d){
return "X" + (d.index +1)
});
inputGroups.moveUp();
};
shallowNeuralNetworkGrapher.prototype.graphWeightNodes = function(data){
var radius = this.nodeRadius * 0.6;
var t = d3.transition()
.duration(750);
// JOIN
var groupElements = this.neuralNetworkG.selectAll(this.svgElement + " .weight-group" )
.data(data);
// EXIT old elements not present in new data.
groupElements.exit()
.attr("class", "exit")
.remove();
// UPDATE old elements present in new data.
groupElements.attr("class", "weight-group")
.attr("transform", function(d){
return "translate(" +
(d.x)
+ ","
+ (d.y) + ")";
});
groupElements.select("#weight0Value")
.text(function(d){
return "W" + (d.outputIndex + 1) + "," + (d.inputIndex + 1);
});
// ENTER new elements present in new data.
var groups = groupElements.enter()
.append("g")
.attr("class", "weight-group")
.attr("transform", function(d){
return "translate(" +
(d.x)
+ ","
+ (d.y) + ")";
});
groups
.append("ellipse")
.attr("class", "weightNode")
.attr("rx", radius * this.weightNodeWidthRatio)
.attr("ry", radius)
.attr("cx", 0)
.attr("cy", 0)
.transition(t)
.attr("y", 0)
.style("fill-opacity", 1);
groups.append("text")
.attr("id", "weight0Value")
.attr("class", "grapherWeightNodeText")
.attr("text-anchor", "middle")
.attr("y", 3)
.text(function(d){
return "W" + (d.outputIndex + 1) + "," + (d.inputIndex + 1);
});
groupElements.moveUp();
};
shallowNeuralNetworkGrapher.prototype.graphBiasNodes = function (data) {
// JOIN
var biasNodes = this.neuralNetworkG.selectAll(this.svgElement + " .bias-group" )
.data(data);
// EXIT old elements not present in new data.
biasNodes.exit()
.attr("class", "exit")
.remove();
// UPDATE old elements present in new data.
biasNodes.attr("class", "bias-group")
.attr("transform", function(d){
return "translate(" +
(d.x)
+ ","
+ (d.y - this.nodeRadius) + ")";
}.bind(this));
biasNodes.moveUp();
// ENTER new elements present in new data.
var biases = biasNodes.enter()
.append("g")
.attr("class", "bias-group")
.attr("transform", function(d){
return "translate(" +
(d.x)
+ ","
+ (d.y - this.nodeRadius) + ")";
}.bind(this));
biases
.append("rect")
.attr("class", "biasNode")
.attr("width", this.nodeRadius * 2)
.attr("height", this.nodeRadius * 2)
.attr("rx", this.nodeRadius / 4)
.attr("ry", this.nodeRadius / 4)
.attr("x", 0)
.attr("y", 0);
biases.append("text")
.attr("id", "biasValue")
.attr("class", "grapher-node-text")
.attr("text-anchor", "middle")
.attr("x", this.nodeRadius)
.attr("y", this.nodeRadius + 5)
.text(function(d){
return "+b" + (d.index +1)
});
};
shallowNeuralNetworkGrapher.prototype.graphOutputNodes = function (data) {
// JOIN
var inputGroups = this.neuralNetworkG.selectAll(this.svgElement + " .output-group" )
.data(data);
// EXIT old elements not present in new data.
inputGroups.exit()
.attr("class", "exit")
.remove();
// UPDATE old elements present in new data.
inputGroups.attr("class", "output-group")
.attr("transform", function(d){
return "translate(" +
(d.x)
+ ","
+ (d.y) + ")";
}.bind(this));
inputGroups.select("#output-name")
.text(function(d){
return "Y" + (d.index +1)
});
// ENTER new elements present in new data.
var inputs = inputGroups.enter()
.append("g")
.attr("class", "output-group")
.attr("transform", function(d){
return "translate(" +
(d.x)
+ ","
+ (d.y) + ")";
}.bind(this));
inputs
.append("circle")
.attr("class", "output-node")
.attr("r", this.nodeRadius)
.attr("cx", 0)
.attr("cy", 0);
inputs.append("text")
.attr("id", "output-name")
.attr("class", "grapher-node-text")
.attr("text-anchor", "middle")
.attr("x", 0)
.attr("y", 5)
.text(function(d){
return "Y" + (d.index +1)
});
//var outputNodes = this.neuralNetworkG.selectAll(this.svgElement + " .output-node" )
// .data(data);
//
//outputNodes.exit()
// .remove();
//
//outputNodes
// .attr("cx", function(d){return d.x})
// .attr("cy", function(d){return d.y});
//
//outputNodes.enter()
// .append("circle")
// .attr("class", "output-node")
// .attr("r", this.nodeRadius)
// .attr("cx", function(d){return d.x})
// .attr("cy", function(d){return d.y});
};
shallowNeuralNetworkGrapher.prototype.graphInputToBiasLines = function (data) {
var inputToBiasLines = this.neuralNetworkG.selectAll(this.svgElement + " .input-to-bias-line" )
.data(data);
inputToBiasLines.exit()
.remove();
inputToBiasLines
.attrs({
class: "arrow input-to-bias-line",
x1: function(d){ return d.x1}, // From the center of the input node
y1: function(d){ return d.y1},
x2: function(d){ return d.x2}, // To the center of the bias node
y2: function(d){ return d.y2}
});
inputToBiasLines.enter()
.append('line')
.attrs({
"class": "arrow input-to-bias-line",
"x1": function(d){ return d.x1}, // From the center of the input node
"y1": function(d){ return d.y1},
"x2": function(d){ return d.x2}, // To the center of the bias node
"y2": function(d){ return d.y2}
});
};
shallowNeuralNetworkGrapher.prototype.graphBiasToSoftmaxArrows = function (data) {
var inputToBiasLines = this.neuralNetworkG.selectAll(this.svgElement + " .bias-to-softmax-line" )
.data(data);
inputToBiasLines.exit()
.remove();
inputToBiasLines
.attrs({
class: "arrow bias-to-softmax-line",
"marker-end": "url(#arrow)",
x1: function(d){ return d.x1}, // From the center of the input node
y1: function(d){ return d.y1},
x2: function(d){ return d.x2}, // To the center of the bias node
y2: function(d){ return d.y2}
});
inputToBiasLines.enter()
.append('line')
.attrs({
"class": "arrow bias-to-softmax-line",
"marker-end": "url(#arrow)",
"x1": function(d){ return d.x1}, // From the center of the input node
"y1": function(d){ return d.y1},
"x2": function(d){ return d.x2}, // To the center of the bias node
"y2": function(d){ return d.y2}
});
};
shallowNeuralNetworkGrapher.prototype.graphSoftmaxToOutputArrows = function (data) {
var inputToBiasLines = this.neuralNetworkG.selectAll(this.svgElement + " .softmax-to-output-line" )
.data(data);
inputToBiasLines.exit()
.remove();
inputToBiasLines
.attrs({
class: "arrow softmax-to-output-line",
"marker-end": "url(#arrow)",
x1: function(d){ return d.x1}, // From the center of the input node
y1: function(d){ return d.y1},
x2: function(d){ return d.x2}, // To the center of the bias node
y2: function(d){ return d.y2}
});
inputToBiasLines.enter()
.append('line')
.attrs({
"class": "arrow softmax-to-output-line",
"marker-end": "url(#arrow)",
"x1": function(d){ return d.x1}, // From the center of the input node
"y1": function(d){ return d.y1},
"x2": function(d){ return d.x2}, // To the center of the bias node
"y2": function(d){ return d.y2}
});
};
shallowNeuralNetworkGrapher.prototype.graphSoftmax = function (data) {
var softmax = this.neuralNetworkG.selectAll(this.svgElement + " .softmax" )
.data([data]);
var softmaxGroup = softmax.enter()
.append("g")
.attr("class", "softmax")
.attr("transform", function(d){
return "translate(" +
(d.x)
+ ","
+ (d.y) + ")";
});
softmaxGroup
.append("rect")
.attr("class", "softmaxNode")
.attr("width", this.nodeRadius * 2)
.attr("height", this.neuralNetworkHeight)
.attr("rx", this.nodeRadius / 4)
.attr("ry", this.nodeRadius / 4)
.attr("x", 0-this.nodeRadius/2)
.attr("y", 0);
softmaxGroup.append("text")
.attr("id", "biasValue")
.attr("text-anchor", "middle")
.attr("x", this.neuralNetworkHeight /2)
.attr("y", -2)
.attr("transform", "rotate(90)")
.text("softmax");
};
var softmaxNNExample = new shallowNeuralNetworkGrapher(2, 2, "#shallow-neural-network-graph",
"Basics of Neural Networks - Viz 4 Features & Classes");
//softmaxNNExample.updateNodeCount("input", 4)
//plugin bootstrap minus and plus
// http://bootsnipp.com/snippets/1Pj1d
//http://jsfiddle.net/laelitenetwork/puJ6G/
$( document ).ready(function() {
$('.btn-number').click(function(e){
e.preventDefault();
var fieldName = $(this).attr('data-field');
var type = $(this).attr('data-type');
var input = $("input[name='"+fieldName+"']");
var currentVal = parseInt(input.val());
if (!isNaN(currentVal)) {
if(type == 'minus') {
var minValue = parseInt(input.attr('min'));
if(!minValue) minValue = 1;
if(currentVal > minValue) {
input.val(currentVal - 1).change();
}
if(parseInt(input.val()) == minValue) {
$(this).attr('disabled', true);
}
} else if(type == 'plus') {
var maxValue = parseInt(input.attr('max'));
if(!maxValue) maxValue = 9999999999999;
if(currentVal < maxValue) {
input.val(currentVal + 1).change();
}
if(parseInt(input.val()) == maxValue) {
$(this).attr('disabled', true);
}
}
} else {
input.val(0);
}
});
$('.input-number').focusin(function(){
$(this).data('oldValue', $(this).val());
});
$('.input-number').change(function() {
var minValue = parseInt($(this).attr('min'));
var maxValue = parseInt($(this).attr('max'));
if(!minValue) minValue = 1;
if(!maxValue) maxValue = 9999999999999;
var valueCurrent = parseInt($(this).val());
var name = $(this).attr('name');
if(valueCurrent >= minValue) {
$(".btn-number[data-type='minus'][data-field='"+name+"']").removeAttr('disabled')
} else {
alert('Sorry, the minimum value was reached');
$(this).val($(this).data('oldValue'));
}
if(valueCurrent <= maxValue) {
$(".btn-number[data-type='plus'][data-field='"+name+"']").removeAttr('disabled')
} else {
alert('Sorry, the maximum value was reached');
$(this).val($(this).data('oldValue'));
}
///var fieldName = $(this).attr('data-field');
var fieldName = $(this).attr('name');
if(fieldName == "quant[1]"){
softmaxNNExample.updateNodeCount("input", valueCurrent);
ga('send', 'event', softmaxNNExample.analyticsCategory, "Set number of", "Features", valueCurrent);
}
else if(fieldName == "quant[2]") {
softmaxNNExample.updateNodeCount("output", valueCurrent);
ga('send', 'event', softmaxNNExample.analyticsCategory, "Set number of", "Classes", valueCurrent);
}
});
$(".input-number").keydown(function (e) {
// Allow: backspace, delete, tab, escape, enter and .
if ($.inArray(e.keyCode, [46, 8, 9, 27, 13, 190]) !== -1 ||
// Allow: Ctrl+A
(e.keyCode == 65 && e.ctrlKey === true) ||
// Allow: home, end, left, right
(e.keyCode >= 35 && e.keyCode <= 39)) {
// let it happen, don't do anything
return;
}
// Ensure that it is a number and stop the keypress
if ((e.shiftKey || (e.keyCode < 48 || e.keyCode > 57)) && (e.keyCode < 96 || e.keyCode > 105)) {
e.preventDefault();
}
});
}); | {
"content_hash": "d762d5fcfbbb72bd54011bbfd22de356",
"timestamp": "",
"source": "github",
"line_count": 667,
"max_line_length": 135,
"avg_line_length": 32.47976011994003,
"alnum_prop": 0.5535912112259971,
"repo_name": "gautham20/gautham20.github.io",
"id": "fbb5312239b2e4eaf7837d69cd10210c4d712bd6",
"size": "21664",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "js/shallow_nn_grapher.js",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "CSS",
"bytes": "165722"
},
{
"name": "HTML",
"bytes": "12305"
},
{
"name": "JavaScript",
"bytes": "484830"
},
{
"name": "Jupyter Notebook",
"bytes": "3891"
}
],
"symlink_target": ""
} |
.storefront-single-product-pagination a {
display: block;
width: 48%; }
.storefront-single-product-pagination a[rel="prev"] {
float: left; }
.storefront-single-product-pagination a[rel="next"] {
float: right; }
@media screen and (min-width: 768px) {
.storefront-single-product-pagination {
z-index: 999999; }
.storefront-single-product-pagination h2 {
clip: rect(1px 1px 1px 1px);
/* IE6, IE7 */
clip: rect(1px, 1px, 1px, 1px);
position: absolute !important; }
.storefront-single-product-pagination a {
display: block;
width: 500px;
position: fixed;
float: none;
background-color: #ffffff;
top: 50%;
z-index: 999999;
box-shadow: 0 0 1em rgba(0, 0, 0, 0.1);
-webkit-transform: translateY(-50%);
-moz-transform: translateY(-50%);
-ms-transform: translateY(-50%);
-o-transform: translateY(-50%);
transform: translateY(-50%);
line-height: 1.2;
overflow: hidden; }
.storefront-single-product-pagination a img {
width: 90px;
border-radius: 0; }
.storefront-single-product-pagination a .title {
width: 340px; }
.storefront-single-product-pagination a[rel="prev"] {
left: -455px;
border-left: 0;
-webkit-transition: left 0.3s ease-out;
-moz-transition: left 0.3s ease-out;
transition: left 0.3s ease-out; }
.storefront-single-product-pagination a[rel="prev"] img {
float: right;
margin-left: 1em; }
.storefront-single-product-pagination a[rel="prev"]:before {
display: block;
font-family: 'FontAwesome';
content: "\f104";
position: absolute;
top: 50%;
left: 1em;
height: 1em;
width: 1em;
-webkit-transform: translateY(-50%);
-moz-transform: translateY(-50%);
-ms-transform: translateY(-50%);
-o-transform: translateY(-50%);
transform: translateY(-50%);
line-height: 1;
text-align: center; }
.storefront-single-product-pagination a[rel="prev"]:hover {
left: 0; }
.storefront-single-product-pagination a[rel="prev"] .title {
display: block;
position: absolute;
right: 110px;
top: 50%;
-webkit-transform: translateY(-50%);
-moz-transform: translateY(-50%);
-ms-transform: translateY(-50%);
-o-transform: translateY(-50%);
transform: translateY(-50%); }
.storefront-single-product-pagination a[rel="next"] {
right: -455px;
border-right: 0;
-webkit-transition: right 0.3s ease-in;
-moz-transition: right 0.3s ease-in;
transition: right 0.3s ease-in; }
.storefront-single-product-pagination a[rel="next"] img {
float: left;
margin-right: 1em; }
.storefront-single-product-pagination a[rel="next"]:hover {
right: 0; }
.storefront-single-product-pagination a[rel="next"]:after {
display: block;
font-family: 'FontAwesome';
content: "\f105";
position: absolute;
top: 50%;
right: 1em;
height: 1em;
width: 1em;
-webkit-transform: translateY(-50%);
-moz-transform: translateY(-50%);
-ms-transform: translateY(-50%);
-o-transform: translateY(-50%);
transform: translateY(-50%);
line-height: 1;
text-align: center; }
.storefront-single-product-pagination a[rel="next"] .title {
display: block;
position: absolute;
left: 110px;
top: 50%;
-webkit-transform: translateY(-50%);
-moz-transform: translateY(-50%);
-ms-transform: translateY(-50%);
-o-transform: translateY(-50%);
transform: translateY(-50%);
text-align: right; } }
| {
"content_hash": "e8a1ba9ae2e33c0dacac8eda85006f31",
"timestamp": "",
"source": "github",
"line_count": 112,
"max_line_length": 68,
"avg_line_length": 35.625,
"alnum_prop": 0.5583959899749373,
"repo_name": "williampansky/pants",
"id": "8eb66621aababeabba16ba468b622404a4e557ca",
"size": "3990",
"binary": false,
"copies": "2",
"ref": "refs/heads/master",
"path": "static/projects/illusiveapparel.website/demo/wp-content/plugins/storefront-product-pagination/assets/css/style.css",
"mode": "33261",
"license": "mit",
"language": [
{
"name": "CSS",
"bytes": "658862"
},
{
"name": "HTML",
"bytes": "19377591"
},
{
"name": "Hack",
"bytes": "2495"
},
{
"name": "JavaScript",
"bytes": "317984"
}
],
"symlink_target": ""
} |
'use strict';
var minilog = require('minilog')
, log = minilog('traverson');
/*
* This transform is meant to be run at the very end of a get/post/put/patch/
* delete call. It just extracts the last response from the step and calls
* t.callback with it.
*/
module.exports = function extractDoc(t) {
log.debug('walker.walk has finished');
/*
TODO Breaks a lot of tests although it seems to make perfect sense?!?
if (!t.response) {
t.callback(createError('No response available', errors.InvalidStateError));
return false;
}
*/
t.callback(null, t.step.response);
// This is a so called final transform that is only applied at the very end
// and it always calls t.callback - in contrast to other transforms it does
// not call t.callback in the error case, but as a success.
// We return false to make sure processing ends here.
return false;
};
| {
"content_hash": "038bf17fc6252a5701be0caefb9aafae",
"timestamp": "",
"source": "github",
"line_count": 28,
"max_line_length": 79,
"avg_line_length": 31.571428571428573,
"alnum_prop": 0.6990950226244343,
"repo_name": "traverson/traverson",
"id": "1a8345ff39338d4373d42de93cec1da73e8e0d45",
"size": "884",
"binary": false,
"copies": "5",
"ref": "refs/heads/master",
"path": "lib/transforms/extract_response.js",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "HTML",
"bytes": "6064"
},
{
"name": "JavaScript",
"bytes": "214314"
},
{
"name": "Shell",
"bytes": "2874"
}
],
"symlink_target": ""
} |
#ifndef __SYSTEMC_EXT_UTIL_SC_REPORT_HANDLER_HH__
#define __SYSTEMC_EXT_UTIL_SC_REPORT_HANDLER_HH__
#include <string>
#include "messages.hh"
#include "sc_report.hh" // for sc_severity
namespace sc_core
{
typedef unsigned sc_actions;
enum
{
SC_UNSPECIFIED = 0x0000,
SC_DO_NOTHING = 0x0001,
SC_THROW = 0x0002,
SC_LOG = 0x0004,
SC_DISPLAY = 0x0008,
SC_CACHE_REPORT = 0x0010,
SC_INTERRUPT = 0x0020,
SC_STOP = 0x0040,
SC_ABORT = 0x0080,
// The spec says these should be macros, but that breaks the build for the
// regression tests since they refer to, for instance,
// sc_core::SC_DEFAULT_INFO_ACTIONS.
SC_DEFAULT_INFO_ACTIONS = SC_LOG | SC_DISPLAY,
SC_DEFAULT_WARNING_ACTIONS = SC_LOG | SC_DISPLAY,
SC_DEFAULT_ERROR_ACTIONS = SC_LOG | SC_CACHE_REPORT | SC_THROW,
SC_DEFAULT_FATAL_ACTIONS = SC_LOG | SC_DISPLAY | SC_CACHE_REPORT | SC_ABORT
};
typedef void (*sc_report_handler_proc)(const sc_report &, const sc_actions &);
class sc_report_handler
{
public:
static void report(sc_severity, const char *msg_type, const char *msg,
const char *file, int line);
static void report(sc_severity, const char *msg_type, const char *msg,
int verbosity, const char *file, int line);
// Deprecated
static void report(sc_severity, int id, const char *msg, const char *file,
int line);
static sc_actions set_actions(sc_severity, sc_actions=SC_UNSPECIFIED);
static sc_actions set_actions(const char *msg_type,
sc_actions=SC_UNSPECIFIED);
static sc_actions set_actions(const char *msg_type, sc_severity,
sc_actions=SC_UNSPECIFIED);
static int stop_after(sc_severity, int limit=-1);
static int stop_after(const char *msg_type, int limit=-1);
static int stop_after(const char *msg_type, sc_severity, int limit=-1);
static int get_count(sc_severity);
static int get_count(const char *msg_type);
static int get_count(const char *msg_type, sc_severity);
// Nonstandard
// In the spec, these aren't listed as static functions. They are static in
// the Accellera implementation and are used as such in the tests.
static int set_verbosity_level(int);
static int get_verbosity_level();
static sc_actions suppress(sc_actions);
static sc_actions suppress();
static sc_actions force(sc_actions);
static sc_actions force();
static sc_actions set_catch_actions(sc_actions);
static sc_actions get_catch_actions();
static void set_handler(sc_report_handler_proc);
static void default_handler(const sc_report &, const sc_actions &);
static sc_actions get_new_action_id();
static sc_report *get_cached_report();
static void clear_cached_report();
static bool set_log_file_name(const char *);
static const char *get_log_file_name();
};
#define SC_REPORT_INFO_VERB(msg_type, msg, verbosity) \
::sc_core::sc_report_handler::report( \
::sc_core::SC_INFO, msg_type, msg, verbosity, __FILE__, __LINE__)
#define SC_REPORT_INFO(msg_type, msg) \
::sc_core::sc_report_handler::report( \
::sc_core::SC_INFO, msg_type, msg, __FILE__, __LINE__)
#define SC_REPORT_WARNING(msg_type, msg) \
::sc_core::sc_report_handler::report( \
::sc_core::SC_WARNING, msg_type, msg, __FILE__, __LINE__)
#define SC_REPORT_ERROR(msg_type, msg) \
::sc_core::sc_report_handler::report( \
::sc_core::SC_ERROR, msg_type, msg, __FILE__, __LINE__)
#define SC_REPORT_FATAL(msg_type, msg) \
::sc_core::sc_report_handler::report( \
::sc_core::SC_FATAL, msg_type, msg, __FILE__, __LINE__)
#define sc_assert(expr) \
((void)((expr) ? 0 : (SC_REPORT_FATAL( \
::sc_core::SC_ID_ASSERTION_FAILED_, #expr), 0)))
void sc_interrupt_here(const char *msg_type, sc_severity);
void sc_stop_here(const char *msg_type, sc_severity);
// Nonstandard
// From Accellera, "not documented, but available".
const std::string sc_report_compose_message(const sc_report &);
bool sc_report_close_default_log();
} // namespace sc_core
#endif //__SYSTEMC_EXT_UTIL_SC_REPORT_HANDLER_HH__
| {
"content_hash": "2f96bfc7d64375a7189ad7f20c1bad20",
"timestamp": "",
"source": "github",
"line_count": 124,
"max_line_length": 79,
"avg_line_length": 34.33064516129032,
"alnum_prop": 0.6408268733850129,
"repo_name": "TUD-OS/gem5-dtu",
"id": "a3bf314bbe940f60fdd5929d89051f8ee3c0e006",
"size": "5804",
"binary": false,
"copies": "5",
"ref": "refs/heads/dtu-mmu",
"path": "src/systemc/ext/utils/sc_report_handler.hh",
"mode": "33188",
"license": "bsd-3-clause",
"language": [
{
"name": "Assembly",
"bytes": "648342"
},
{
"name": "Awk",
"bytes": "3386"
},
{
"name": "C",
"bytes": "1717604"
},
{
"name": "C++",
"bytes": "35149040"
},
{
"name": "CMake",
"bytes": "79529"
},
{
"name": "Emacs Lisp",
"bytes": "1969"
},
{
"name": "Forth",
"bytes": "15790"
},
{
"name": "HTML",
"bytes": "136898"
},
{
"name": "Java",
"bytes": "3179"
},
{
"name": "M4",
"bytes": "75007"
},
{
"name": "Makefile",
"bytes": "68265"
},
{
"name": "Objective-C",
"bytes": "24714"
},
{
"name": "Perl",
"bytes": "33696"
},
{
"name": "Python",
"bytes": "6073714"
},
{
"name": "Roff",
"bytes": "8783"
},
{
"name": "SWIG",
"bytes": "173"
},
{
"name": "Scala",
"bytes": "14236"
},
{
"name": "Shell",
"bytes": "101649"
},
{
"name": "VBA",
"bytes": "2884"
},
{
"name": "Vim Script",
"bytes": "4335"
},
{
"name": "sed",
"bytes": "3927"
}
],
"symlink_target": ""
} |
def welcome string
if string.include? "CA"
p "Welcome to California"
else
p "You should move to California"
end
end | {
"content_hash": "4dfbf0832e3457880db35020a3112294",
"timestamp": "",
"source": "github",
"line_count": 7,
"max_line_length": 35,
"avg_line_length": 17.571428571428573,
"alnum_prop": 0.7235772357723578,
"repo_name": "ckLee8/phase-0",
"id": "33b64dc381254b4523c9d2ba10bbb1ba48422226",
"size": "218",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "week-4/simple-substrings/my_solution.rb",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "CSS",
"bytes": "2902"
},
{
"name": "HTML",
"bytes": "13140"
},
{
"name": "JavaScript",
"bytes": "28586"
},
{
"name": "Ruby",
"bytes": "106449"
}
],
"symlink_target": ""
} |
#import "TiProxy.h"
#import "TiAnimation.h"
#import "TiGradient.h"
#import "LayoutConstraint.h"
//By declaring a scrollView protocol, TiUITextWidget can access
@class TiUIView;
/**
The protocol for scrolling.
*/
@protocol TiScrolling
/**
Tells the scroll view that keyboard did show.
@param keyboardTop The keyboard height.
*/
-(void)keyboardDidShowAtHeight:(CGFloat)keyboardTop;
/**
Tells the scroll view to scroll to make the specified view visible.
@param firstResponderView The view to make visible.
@param keyboardTop The keyboard height.
*/
-(void)scrollToShowView:(TiUIView *)firstResponderView withKeyboardHeight:(CGFloat)keyboardTop;
@end
void InsetScrollViewForKeyboard(UIScrollView * scrollView,CGFloat keyboardTop,CGFloat minimumContentHeight);
void OffsetScrollViewForRect(UIScrollView * scrollView,CGFloat keyboardTop,CGFloat minimumContentHeight,CGRect responderRect);
void ModifyScrollViewForKeyboardHeightAndContentHeightWithResponderRect(UIScrollView * scrollView,CGFloat keyboardTop,CGFloat minimumContentHeight,CGRect responderRect);
@class TiViewProxy;
/**
Base class for all notice_view views.
@see TiViewProxy
*/
@interface TiUIView : UIView<TiProxyDelegate,LayoutAutosizing>
{
@protected
BOOL configurationSet;
@private
TiProxy *proxy;
TiAnimation *animation;
CALayer *gradientLayer;
CGAffineTransform virtualParentTransform;
id transformMatrix;
BOOL childrenInitialized;
BOOL touchEnabled;
unsigned int animationDelayGuard;
// Touch detection
BOOL changedInteraction;
BOOL handlesTouches;
UIView *touchDelegate; // used for touch delegate forwarding
BOOL animating;
UITapGestureRecognizer* singleTapRecognizer;
UITapGestureRecognizer* doubleTapRecognizer;
UITapGestureRecognizer* twoFingerTapRecognizer;
UIPinchGestureRecognizer* pinchRecognizer;
UISwipeGestureRecognizer* leftSwipeRecognizer;
UISwipeGestureRecognizer* rightSwipeRecognizer;
UILongPressGestureRecognizer* longPressRecognizer;
//Resizing handling
CGSize oldSize;
// Image capping/backgrounds
id backgroundImage;
BOOL backgroundRepeat;
TiDimension leftCap;
TiDimension topCap;
}
/**
Returns current status of the view animation.
@return _YES_ if view is being animated, _NO_ otherwise.
*/
-(BOOL)animating;
/**
Provides access to a proxy object of the view.
*/
@property(nonatomic,readwrite,assign) TiProxy *proxy;
/**
Provides access to touch delegate of the view.
Touch delegate is the control that receives all touch events.
*/
@property(nonatomic,readwrite,assign) UIView *touchDelegate;
/**
Returns view's transformation matrix.
*/
@property(nonatomic,readonly) id transformMatrix;
/**
Provides access to background image of the view.
*/
@property(nonatomic,readwrite,retain) id backgroundImage;
/**
Returns enablement of touch events.
@see updateTouchHandling
*/
@property(nonatomic,readonly) BOOL touchEnabled;
@property(nonatomic,readonly) UITapGestureRecognizer* singleTapRecognizer;
@property(nonatomic,readonly) UITapGestureRecognizer* doubleTapRecognizer;
@property(nonatomic,readonly) UITapGestureRecognizer* twoFingerTapRecognizer;
@property(nonatomic,readonly) UIPinchGestureRecognizer* pinchRecognizer;
@property(nonatomic,readonly) UISwipeGestureRecognizer* leftSwipeRecognizer;
@property(nonatomic,readonly) UISwipeGestureRecognizer* rightSwipeRecognizer;
@property(nonatomic,readonly) UILongPressGestureRecognizer* longPressRecognizer;
-(void)configureGestureRecognizer:(UIGestureRecognizer*)gestureRecognizer;
-(UIGestureRecognizer *)gestureRecognizerForEvent:(NSString *)event;
/**
Returns CA layer for the background of the view.
*/
-(CALayer *)backgroundImageLayer;
/**
Tells the view to start specified animation.
@param newAnimation The animation to start.
*/
-(void)animate:(TiAnimation *)newAnimation;
#pragma mark Framework
/**
Performs view's initialization procedure.
*/
-(void)initializeState;
/**
Performs view's configuration procedure.
*/
-(void)configurationSet;
/**
Sets virtual parent transformation for the view.
@param newTransform The transformation to set.
*/
-(void)setVirtualParentTransform:(CGAffineTransform)newTransform;
-(void)setTransform_:(id)matrix;
/*
Tells the view to load an image.
@param image The string referring the image.
@return The loaded image.
*/
-(UIImage*)loadImage:(id)image;
-(id)proxyValueForKey:(NSString *)key;
-(void)readProxyValuesWithKeys:(id<NSFastEnumeration>)keys;
/*
Tells the view to change its proxy to the new one provided.
@param newProxy The new proxy to set on the view.
*/
-(void)transferProxy:(TiViewProxy*)newProxy;
/**
Tells the view to update its touch handling state.
@see touchEnabled
*/
-(void)updateTouchHandling;
/**
Tells the view that its frame and/or bounds has chnaged.
@param frame The frame rect
@param bounds The bounds rect
*/
-(void)frameSizeChanged:(CGRect)frame bounds:(CGRect)bounds;
/**
Tells the view to make its root view a first responder.
*/
-(void)makeRootViewFirstResponder;
-(void)animationCompleted;
/**
The convenience method to raise an exception for the view.
@param reason The exception reason.
@param subreason The exception subreason.
@param location The exception location.
*/
+(void)throwException:(NSString *) reason subreason:(NSString*)subreason location:(NSString *)location;
-(void)throwException:(NSString *) reason subreason:(NSString*)subreason location:(NSString *)location;
/**
Returns default enablement for interactions.
Subclasses may override.
@return _YES_ if the control has interactions enabled by default, _NO_ otherwise.
*/
-(BOOL)interactionDefault;
-(BOOL)interactionEnabled;
/**
Whether or not the view has any touchable listeners attached.
@return _YES_ if the control has any touchable listener attached, _NO_ otherwise.
*/
-(BOOL)hasTouchableListener;
-(void)handleControlEvents:(UIControlEvents)events;
-(void)setVisible_:(id)visible;
-(UIView *)gradientWrapperView;
-(void)checkBounds;
- (void)processTouchesBegan:(NSSet *)touches withEvent:(UIEvent *)event;
- (void)processTouchesMoved:(NSSet *)touches withEvent:(UIEvent *)event;
- (void)processTouchesEnded:(NSSet *)touches withEvent:(UIEvent *)event;
- (void)processTouchesCancelled:(NSSet *)touches withEvent:(UIEvent *)event;
@end
#pragma mark TO REMOVE, used only during transition.
#define USE_PROXY_FOR_METHOD(resultType,methodname,inputType) \
-(resultType)methodname:(inputType)value \
{ \
NSLog(@"[DEBUG] Using view proxy via redirection instead of directly for %@.",self); \
return [(TiViewProxy *)[self proxy] methodname:value]; \
}
#define USE_PROXY_FOR_VERIFY_AUTORESIZING USE_PROXY_FOR_METHOD(UIViewAutoresizing,verifyAutoresizing,UIViewAutoresizing)
| {
"content_hash": "f4fdb15cb5d88bb57ada2d6821ebe8c4",
"timestamp": "",
"source": "github",
"line_count": 245,
"max_line_length": 169,
"avg_line_length": 27.612244897959183,
"alnum_prop": 0.7806356245380636,
"repo_name": "vanbungkring/ios-NoticeView",
"id": "0704dd980dc462391bb6f2c0e3d6bbcae6a2dac4",
"size": "7087",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "build/iphone/Classes/TiUIView.h",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "C",
"bytes": "181808"
},
{
"name": "C++",
"bytes": "47528"
},
{
"name": "D",
"bytes": "1176323"
},
{
"name": "JavaScript",
"bytes": "8751"
},
{
"name": "Objective-C",
"bytes": "3216119"
},
{
"name": "Shell",
"bytes": "304"
}
],
"symlink_target": ""
} |
I am a software developer currently based in London, UK. Most of my early work experience was centered around server-side development, however the projects I have been involved in since have dealt with everything from UI design to communication protocol implementation. While I would still consider backend development as my core competence, for all intents and purposes I am a full-stack developer.
Outside of programming (which I tend to tinker with in my spare time as well), I am a keen walker, cyclist and (albeit rusty) basketball player. I am also keen consumer of the literary arts and consider a long walk in the company of an audiobook to be an excellent way of spending a Saturday afternoon (as long as the rain does not go above a drizzle).
## experience
#### [LMAX Exchange](https://www.lmax.com) (October 2014 - Present)
LMAX Exchange is a multilateral trading facility (MTF) working in the FX market and that relies and invests heavily in its technology department in order to provide its products.
As part of the development team, my responsibilities include implementing new features (as dictated by business needs), developing new tools to help internal users (task automation, system monitoring etc) and supporting our products (including participating in software releases, addressing tech-related concerns from customers or internal users etc.).
Most of our technology is written in Java and we tend to develop a lot of tooling in-house, as more often than not no off-the-shelf products match our requirements. All the tools and software we write is extensively tested in our CI systems.
#### [DSPG Ltd](http://www.dspg.co.uk) (August 2011 - August 2014)
DSPG Ltd is a technology company specialising in live-text communication, providing call-centre like solutions for companies and organisations and enabling them to better interact with their speech and hearing impaired clients.
As one of the main developers in the company, I was tasked both with maintaining and improving our existing systems and with designing and developing new ones. I provided support for integrating of company products and, on occasion, I travelled on site to install systems and train clients on their usage.
Most of the current technology is based on Java, with Android and iOS mobile application and some older products written in .Net and C/C++.
#### [Freelance Software Developer](http://www.mkcz.net) (Intermittent, Ongoing)
When the opportunity arises, I have also undertaken work as a freelancer. The projects I have been involved in include Android, website and client-server development.
## projects
#### WebSocket Client and Translator Server
_Goal_:
Create a new web-client for an existing system, replacing the old Java Applet one.
_Tasks:_
+ Implement the new client based on the behaviour and design of the current one
+ Design and develop a translator service that would take the new client WebSocket-based messages and convert them to the existing communication protocol (and vice-versa)
_Details:_
The client is based on the design and behaviour of the existing one, but written in HTML5 and JavaScript, with a custom jQuery plugin handling the UI interaction and network communication.
The translator server is written in Java, with Netty handling the WebSocket integration.
I also wrote a backend simulator that, in conjunction with Selenium, allowed for more complete testing of the client and translator.
#### Text Relay Service
_Goal_:
Create a new text relay system meant to replace the existing one, adding new functionality and providing users with easier access to it via PC and mobile applications.
_Tasks_:
+ help in preparing the project specification and presenting it to the client
+ develop one of the PC applications and the main Internet access point for the system
_Details_:
Development was done in Java and included creating a distributed system that would provide almost constant uptime to customers, handling connections between the different parties and implementing a silent recovery method that would provide users with an uninterrupted experience across short connection breakages (such as a mobile user temporarily loosing reception).
#### Electronic fax management system
_Goal_:
Develop an electronic system to help ease client usage of the existing fax system.
_Tasks_:
+ help prepare project specification
+ develop the PC application that would allow users to send faxes
+ install system on client site
_Details_:
Using .Net, I implemented the a client application that would allow users to "print" faxes to the system in order to have them sent on to the desired destination.
#### Android client for existing chat system
_Goal_:
Create an Android client to replace the existing J2ME one.
_Tasks_:
+ understand the communications protocol used by the current client and the interaction in had with the backend service
+ develop an Android client that provided the same behaviour and worked on the same protocol
+ develop a centralised configuration system
_Details_:
My first out-of-university project, it involved me learning both the company technology (the J2ME client and other proprietary Java software) and the Android ecosystem in order to complete it.
Furthermore, I had to develop a centralised way of configuring the clients, to avoid having to release a new version every time something needed updating. I used an Sqlite database to hold the information needed and configured the Android applications to connect to it in order to the information necessary. If the version of the data on the app matched that on the server, a full download was skipped.
#### [Central European Games Conference (2015)](http://www.cegconf.com/2015/)
_Goal_:
Build a website with pertinent details for a gaming conference in Vienna, Austria.
_Tasks_:
+ collaborate with another remote developer to create the website
+ liaise with the designer to ensure our work matched specification
_Details_:
A volunteer project to help with the setting up of the website. We used HTML5 and SASS for most of the work, implementing the design provided, and JavaScript to dynamically load those parts of the site that were more often subject to change (such as team structures).
## tools of the trade
| working knowledge | basic knowledge
:--:|:--:|:--:
techstack|Java SE, MySQL, JavaScript, HTML, bash|Android, Python, C/C++, .Net
software|Linux, IntelliJ Idea|Vim, Windows | {
"content_hash": "92096200cd1a6535e776253b0edaca6e",
"timestamp": "",
"source": "github",
"line_count": 117,
"max_line_length": 402,
"avg_line_length": 55.51282051282051,
"alnum_prop": 0.7881447267128561,
"repo_name": "mkcz/mkcz.net",
"id": "4fff85b34186eb94e42fb8ad02a274f88d96145d",
"size": "6590",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "cv.md",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "CSS",
"bytes": "143618"
},
{
"name": "HTML",
"bytes": "22890"
},
{
"name": "JavaScript",
"bytes": "63595"
}
],
"symlink_target": ""
} |
#ifdef USE_TI_MEDIA
#import "TiMediaAudioPlayerProxy.h"
#import "TiUtils.h"
#import "TiMediaAudioSession.h"
#include <AudioToolbox/AudioToolbox.h>
@implementation TiMediaAudioPlayerProxy
#pragma mark Internal
-(void)_initWithProperties:(NSDictionary *)properties
{
url = [[TiUtils toURL:[properties objectForKey:@"url"] proxy:self] retain];
int initialMode = [TiUtils intValue:@"audioSessionMode"
properties:properties
def:0];
if (initialMode) {
[self setAudioSessionMode:[NSNumber numberWithInt:initialMode]];
}
}
-(void)_destroy
{
if (timer!=nil)
{
[timer invalidate];
RELEASE_TO_NIL(timer);
}
if (player!=nil)
{
if ([player isPlaying] || [player isPaused] || [player isWaiting]) {
[player stop];
[[TiMediaAudioSession sharedSession] stopAudioSession];
}
}
[player setDelegate:nil];
RELEASE_TO_NIL(player);
RELEASE_TO_NIL(timer);
[super _destroy];
}
-(void)_listenerAdded:(NSString *)type count:(int)count
{
if (count == 1 && [type isEqualToString:@"progress"])
{
progress = YES;
}
}
-(void)_listenerRemoved:(NSString *)type count:(int)count
{
if (count == 0 && [type isEqualToString:@"progress"])
{
progress = NO;
}
}
-(AudioStreamer*)player
{
if (player==nil)
{
if (url==nil)
{
[self throwException:@"invalid url" subreason:@"url has not been set" location:CODELOCATION];
}
player = [[AudioStreamer alloc] initWithURL:url];
[player setDelegate:self];
[player setBufferSize:bufferSize];
if (progress)
{
// create progress callback timer that fires once per second. we might want to eventually make this
// more configurable but for now that's sufficient for most apps that want to display progress updates on the stream
timer = [[NSTimer scheduledTimerWithTimeInterval:1 target:self selector:@selector(updateProgress:) userInfo:nil repeats:YES] retain];
}
}
return player;
}
-(void)destroyPlayer
{
if (timer!=nil)
{
[timer invalidate];
RELEASE_TO_NIL(timer);
}
if (player!=nil)
{
if ([player isPlaying] || [player isPaused] || [player isWaiting])
{
[player stop];
[[TiMediaAudioSession sharedSession] stopAudioSession];
}
[player setDelegate:nil];
RELEASE_TO_NIL(player);
}
}
-(void)restart:(id)args
{
BOOL playing = [player isPlaying] || [player isPaused] || [player isWaiting];
[self destroyPlayer];
if (playing)
{
[[self player] start];
}
else
{
// just create it
[self player];
}
}
#pragma mark Public APIs
-(void)setPaused:(NSNumber *)paused
{
if (player!=nil)
{
if ([TiUtils boolValue:paused])
{
[player pause];
}
else
{
[player start];
}
}
}
#define PLAYER_PROP_BOOL(name,func) \
-(NSNumber*)name\
{\
if (player==nil)\
{\
return NUMBOOL(NO);\
}\
return NUMBOOL([player func]);\
}
#define PLAYER_PROP_DOUBLE(name,func) \
-(NSNumber*)name\
{\
if (player==nil)\
{\
return NUMDOUBLE(0);\
}\
return NUMDOUBLE([player func]);\
}
PLAYER_PROP_BOOL(waiting,isWaiting);
PLAYER_PROP_BOOL(idle,isIdle);
PLAYER_PROP_BOOL(playing,isPlaying);
PLAYER_PROP_BOOL(paused,isPaused);
PLAYER_PROP_DOUBLE(bitRate,bitRate);
PLAYER_PROP_DOUBLE(progress,progress);
PLAYER_PROP_DOUBLE(state,state);
-(void)setBufferSize:(NSNumber*)bufferSize_
{
bufferSize = [bufferSize_ unsignedIntegerValue];
if (player != nil) {
[player setBufferSize:bufferSize];
}
}
-(NSNumber*)bufferSize
{
return [NSNumber numberWithUnsignedInteger:((bufferSize) ? bufferSize : kAQDefaultBufSize)];
}
-(void)setUrl:(id)args
{
if (![NSThread isMainThread]) {
TiThreadPerformOnMainThread(^{[self setUrl:args];}, YES);
return;
}
RELEASE_TO_NIL(url);
ENSURE_SINGLE_ARG(args,NSString);
url = [[TiUtils toURL:args proxy:self] retain];
if (player!=nil)
{
[self restart:nil];
}
}
-(NSURL*)url
{
return url;
}
// Only need to ensure the UI thread when starting; and we should actually wait until it's finished so
// that execution flow is correct (if we stop/pause immediately after)
-(void)start:(id)args
{
if (![NSThread isMainThread]) {
TiThreadPerformOnMainThread(^{[self start:args];}, YES);
return;
}
// indicate we're going to start playing
if (![[TiMediaAudioSession sharedSession] canPlayback]) {
[self throwException:@"Improper audio session mode for playback"
subreason:[[NSNumber numberWithUnsignedInt:[[TiMediaAudioSession sharedSession] sessionMode]] description]
location:CODELOCATION];
}
if (player == nil || !([player isPlaying] || [player isPaused] || [player isWaiting])) {
[[TiMediaAudioSession sharedSession] startAudioSession];
}
[[self player] start];
}
-(void)stop:(id)args
{
if (![NSThread isMainThread]) {
TiThreadPerformOnMainThread(^{[self stop:args];}, YES);
return;
}
if (player!=nil)
{
if ([player isPlaying] || [player isPaused] || [player isWaiting])
{
[player stop];
[[TiMediaAudioSession sharedSession] stopAudioSession];
}
}
}
-(void)pause:(id)args
{
if (![NSThread isMainThread]) {
TiThreadPerformOnMainThread(^{[self pause:args];}, YES);
return;
}
if (player!=nil)
{
[player pause];
}
}
MAKE_SYSTEM_PROP(STATE_INITIALIZED,AS_INITIALIZED);
MAKE_SYSTEM_PROP(STATE_STARTING,AS_STARTING_FILE_THREAD);
MAKE_SYSTEM_PROP(STATE_WAITING_FOR_DATA,AS_WAITING_FOR_DATA);
MAKE_SYSTEM_PROP(STATE_WAITING_FOR_QUEUE,AS_WAITING_FOR_QUEUE_TO_START);
MAKE_SYSTEM_PROP(STATE_PLAYING,AS_PLAYING);
MAKE_SYSTEM_PROP(STATE_BUFFERING,AS_BUFFERING);
MAKE_SYSTEM_PROP(STATE_STOPPING,AS_STOPPING);
MAKE_SYSTEM_PROP(STATE_STOPPED,AS_STOPPED);
MAKE_SYSTEM_PROP(STATE_PAUSED,AS_PAUSED);
-(void)setAudioSessionMode:(NSNumber*)mode
{
UInt32 newMode = [mode unsignedIntegerValue]; // Close as we can get to UInt32
if (newMode == kAudioSessionCategory_RecordAudio) {
NSLog(@"[WARN] Invalid mode for audio player... setting to default.");
newMode = kAudioSessionCategory_SoloAmbientSound;
}
NSLog(@"[WARN] 'TabbedQRExample.Media.AudioPlayer.audioSessionMode' is deprecated; use 'TabbedQRExample.Media.audioSessionMode'");
[[TiMediaAudioSession sharedSession] setSessionMode:newMode];
}
-(NSNumber*)audioSessionMode
{
NSLog(@"[WARN] 'TabbedQRExample.Media.AudioPlayer.audioSessionMode' is deprecated; use 'TabbedQRExample.Media.audioSessionMode'");
return [NSNumber numberWithUnsignedInteger:[[TiMediaAudioSession sharedSession] sessionMode]];
}
-(NSString*)stateToString:(int)state
{
switch(state)
{
case AS_INITIALIZED:
return @"initialized";
case AS_STARTING_FILE_THREAD:
return @"starting";
case AS_WAITING_FOR_DATA:
return @"waiting_for_data";
case AS_WAITING_FOR_QUEUE_TO_START:
return @"waiting_for_queue";
case AS_PLAYING:
return @"playing";
case AS_BUFFERING:
return @"buffering";
case AS_STOPPING:
return @"stopping";
case AS_STOPPED:
return @"stopped";
case AS_PAUSED:
return @"paused";
}
return @"unknown";
}
-(NSString*)stateDescription:(id)arg
{
ENSURE_SINGLE_ARG(arg,NSNumber);
return [self stateToString:[TiUtils intValue:arg]];
}
#pragma mark Delegates
-(void)playbackStateChanged:(id)sender
{
if ([self _hasListeners:@"change"])
{
NSDictionary *event = [NSDictionary dictionaryWithObjectsAndKeys:[self state],@"state",[self stateToString:player.state],@"description",nil];
[self fireEvent:@"change" withObject:event];
}
if (player.errorCode != AS_NO_ERROR && player.state == AS_STOPPED) {
[[TiMediaAudioSession sharedSession] stopAudioSession];
}
}
- (void)updateProgress:(NSTimer *)updatedTimer
{
if (player!=nil && [player isPlaying])
{
double value = 0;
if (player.bitRate != 0.0)
{
value = player.progress;
}
NSDictionary *event = [NSDictionary dictionaryWithObject:NUMDOUBLE(value) forKey:@"progress"];
[self fireEvent:@"progress" withObject:event];
}
}
@end
#endif | {
"content_hash": "f4748f3f8f2b4a47bf397eb08022550a",
"timestamp": "",
"source": "github",
"line_count": 336,
"max_line_length": 143,
"avg_line_length": 23.270833333333332,
"alnum_prop": 0.6958690369612482,
"repo_name": "acktie/Tabbed-QR-Example",
"id": "686ae013e5c4f0a936b43981d2e27e6cc0aa6e42",
"size": "8141",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "build/iphone/Classes/TiMediaAudioPlayerProxy.m",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "C",
"bytes": "156688"
},
{
"name": "C++",
"bytes": "43981"
},
{
"name": "JavaScript",
"bytes": "2894"
},
{
"name": "Objective-C",
"bytes": "3115174"
}
],
"symlink_target": ""
} |
ACCEPTED
#### According to
The Catalogue of Life, 3rd January 2011
#### Published in
null
#### Original name
null
### Remarks
null | {
"content_hash": "3c47ad6d7449eca70724b6ed0e70e6e5",
"timestamp": "",
"source": "github",
"line_count": 13,
"max_line_length": 39,
"avg_line_length": 10.307692307692308,
"alnum_prop": 0.6940298507462687,
"repo_name": "mdoering/backbone",
"id": "40f9c78f38a8619dc4b7a59936aefa023dfc9748",
"size": "210",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "life/Bacteria/Firmicutes/Clostridia/Clostridiales/Peptococcaceae/Desulfotomaculum/Desulfotomaculum aeronauticum/README.md",
"mode": "33188",
"license": "apache-2.0",
"language": [],
"symlink_target": ""
} |
package notebook.kernel
import xml.NodeSeq
/**
* Result of evaluating something in the REPL.
*
* The difference between Incomplete and Failure is Incomplete means
* the expression failed to compile whereas Failure means an exception
* was thrown during executing the code.
*/
sealed abstract class EvaluationResult
case object Incomplete extends EvaluationResult
case class Failure(stackTrace: String) extends EvaluationResult
case class Success(result: NodeSeq) extends EvaluationResult
| {
"content_hash": "da5cd3c26d3a50d40839b6edd38ac29c",
"timestamp": "",
"source": "github",
"line_count": 18,
"max_line_length": 70,
"avg_line_length": 27.72222222222222,
"alnum_prop": 0.8096192384769539,
"repo_name": "bigdatagenomics/mango-notebook",
"id": "f85a77324b3879d77a178d5c05ecdc3f4ae50a99",
"size": "701",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "modules/kernel/src/main/scala/notebook/kernel/EvaluationResult.scala",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "CSS",
"bytes": "256181"
},
{
"name": "CoffeeScript",
"bytes": "5483"
},
{
"name": "Java",
"bytes": "366"
},
{
"name": "JavaScript",
"bytes": "3735335"
},
{
"name": "Scala",
"bytes": "168685"
}
],
"symlink_target": ""
} |
.jchartfx {
font-family: Arial, sans-serif;
font-weight: normal;
font-size: 8pt;
line-height: normal;
}
.jchartfxToolTip {
padding: 3px;
font-size: 8pt;
font-family: Arial, sans-serif;
font-weight: bold;
-moz-border-radius: 3px;
border-radius: 3px;
pointer-events: none;
border-style: solid;
border-width: 1px;
}
.jchartfxToolTipVisible {
opacity: 1;
-webkit-transition: opacity 0.5s ease-in-out;
-moz-transition: opacity 0.5s ease-in-out;
-o-transition: opacity 0.5s ease-in-out;
-ms-transition: opacity 0.5s ease-in-out;
transition: opacity 0.5s ease-in-out;
}
.jchartfxToolTipHidden {
opacity: 0;
-webkit-transition: opacity 0.5s ease-in-out;
-moz-transition: opacity 0.5s ease-in-out;
-o-transition: opacity 0.5s ease-in-out;
-ms-transition: opacity 0.5s ease-in-out;
transition: opacity 0.5s ease-in-out;
}
.jchartfxToolTip2 {
pointer-events: none;
}
.jchartfxToolTip2Visible {
opacity: 1;
-webkit-transition: opacity 0.5s ease-in-out;
-moz-transition: opacity 0.5s ease-in-out;
-o-transition: opacity 0.5s ease-in-out;
-ms-transition: opacity 0.5s ease-in-out;
transition: opacity 0.5s ease-in-out;
}
.jchartfxToolTip2Hidden {
opacity: 0;
-webkit-transition: opacity 0.5s ease-in-out;
-moz-transition: opacity 0.5s ease-in-out;
-o-transition: opacity 0.5s ease-in-out;
-ms-transition: opacity 0.5s ease-in-out;
transition: opacity 0.5s ease-in-out;
}
.jchartfx .Attribute {
stroke-width: 1;
}
.jchartfx .AttributeLine {
stroke-width: 3;
}
.jchartfx .MarkerHollow {
stroke-width: 2;
}
.jchartfx .Marker {
stroke-width: 2;
}
.jchartfx .Border {
stroke-width: 1;
}
.jchartfx .PlotArea {
stroke-width: 1;
}
.jchartfx .PlotArea3D {
stroke-width: 1;
}
.jchartfx .AxisY_Line {
stroke-width: 2;
}
.jchartfx .AxisX_Line {
stroke-width: 2;
}
.jchartfx .Title {
font-size: 10.5pt;
font-weight: bold;
}
.jchartfx .LegendBox {
stroke-width: 1;
}
.jchartfx .ScrollBar {
stroke-width: 1;
}
.jchartfx .EqualizerTop0 {
stroke-width: 1;
}
.jchartfx .EqualizerTop1 {
stroke-width: 1;
}
.jchartfx .GaugeTitle {
font-weight: bold;
font-size: 10.5pt;
}
.jchartfx .Separator {
stroke-width: 1;
}
.jchartfx .MapContext {
stroke-width: 1;
}
.jchartfx .MapWater {
stroke-width: 1;
}
.jchartfx .MapAttribute {
stroke-width: 1;
}
.jchartfx .MapRoad {
stroke-width: 1;
}
.jchartfx .MapRoadBack {
stroke-width: 3;
}
.jchartfx .MapRoad0 {
stroke-width: 1;
}
.jchartfx .MapRoad0Back {
stroke-width: 3;
}
.jchartfx .MapRoad1 {
stroke-width: 1;
}
.jchartfx .MapRoad1Back {
stroke-width: 0;
}
.jchartfx .MapTranslationArea {
stroke-width: 1;
}
.jchartfx .GaugeTitleLarge {
font-size: 40pt;
}
.jchartfx .DashboardTitle {
font-size: 10.5pt;
}
.jchartfx_container, .jchartfx_container *
{
font-family: Arial, sans-serif;
}
.jchartfx_container table
{
border:1px solid;
font-size:8pt;
margin:45px 5%;
width:90%;
border-spacing: 0px;
}
.jchartfx_container table thead tr th
{
border:1px solid;
border-bottom:1px solid;
text-align: left;
padding:5px;
font-weight: normal;
}
.jchartfx_container table tbody tr td
{
padding:5px;
border:1px solid;
}
.jchartfx_container .labelIndicator
{
text-align:center;
margin-top: 50px;
font-size:18.125pt;
line-height:1em;
}
.jchartfx_container .label {
text-align: center;
vertical-align:middle;
font-size: 14pt;
line-height:1em;
}
.jchartfx_container .smallLabel {
font-size: 8pt;
text-align: center;
vertical-align:middle;
line-height:1em;
}
.jchartfx .BorderMedium {
stroke-width: 4;
}
.jchartfx .BorderThick {
stroke-width: 8;
}
.jchartfx .Border0 {
stroke-width: 1;
}
.jchartfx .Border1 {
stroke-width: 1;
}
.jchartfx .Border2 {
stroke-width: 1;
}
.jchartfx .Border3 {
stroke-width: 1;
}
.jchartfx .Border4 {
stroke-width: 1;
}
.jchartfx .Border5 {
stroke-width: 1;
}
.jchartfx .Border6 {
stroke-width: 1;
}
.jchartfx .Border7 {
stroke-width: 1;
} | {
"content_hash": "eec09c1407f5d5498fc0521c0830f396",
"timestamp": "",
"source": "github",
"line_count": 263,
"max_line_length": 49,
"avg_line_length": 16.098859315589355,
"alnum_prop": 0.6414737836561172,
"repo_name": "jenidarnold/racquetball",
"id": "303db15725b3edc10e747d7db97451c2b235e073",
"size": "4234",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "public/css/jchartfx/Attributes/jchartfx.attributes.dot-luv.css",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "ApacheConf",
"bytes": "356"
},
{
"name": "HTML",
"bytes": "414670"
},
{
"name": "JavaScript",
"bytes": "220102"
},
{
"name": "PHP",
"bytes": "293973"
},
{
"name": "Shell",
"bytes": "1490"
}
],
"symlink_target": ""
} |
<?php
$year = date('Y');
$month = date('m');
echo json_encode(array(
array(
'id' => 1,
'title' => "Conservatory Exhibit: The garden of india a country and culture revealed", // Event Title
'start' => "2014-04-04", // Event Start Date
'url' => "event-detail.html" // Event URL
),
array(
'id' => 2,
'title' => "February Half-Term Activities: Big Stars and Little Secrets",
'start' => "$year-$month-10",
'url' => "event-detail.html"
),
array(
'id' => 3,
'title' => "The Orchestra of the Age of Enlightenment perform with Music",
'start' => "$year-$month-11",
'url' => "event-detail.html"
),
array(
'id' => 4,
'title' => "Museums and the Construction of Identities",
'start' => "$year-$month-14",
'url' => "event-detail.html"
),
array(
'id' => 5,
'title' => "Reporting: In Conversation with Miriam Elder and Julia Ioffe",
'start' => "$year-$month-26",
'url' => "event-detail.html"
),
array(
'id' => 5,
'title' => "The Orchestra of the Age of Enlightenment perform with Music",
'start' => "$year-$month-29",
'url' => "event-detail.html"
)
));
?> | {
"content_hash": "a5cb9747cf07d6fba5ddb5ea7e70f8df",
"timestamp": "",
"source": "github",
"line_count": 52,
"max_line_length": 109,
"avg_line_length": 25.78846153846154,
"alnum_prop": 0.48695003728560776,
"repo_name": "nlkhagva/educenter.mn",
"id": "2e18fdeb44eaec2766b950070785ff5f5fbc603e",
"size": "1341",
"binary": false,
"copies": "1",
"ref": "refs/heads/master",
"path": "web/frontend/events.php",
"mode": "33261",
"license": "mit",
"language": [
{
"name": "ASP",
"bytes": "47707"
},
{
"name": "CSS",
"bytes": "1581004"
},
{
"name": "JavaScript",
"bytes": "6037646"
},
{
"name": "PHP",
"bytes": "2261059"
},
{
"name": "Ruby",
"bytes": "208"
},
{
"name": "Shell",
"bytes": "1178"
}
],
"symlink_target": ""
} |
module.exports = function ascii_lowercase () { // eslint-disable-line camelcase
// original by: Yury Shapkarin (https://shapkarin.me)
// example 1: ascii_lowercase()
// returns 1: 'abcdefghijklmnopqrstuvwxyz'
const length = 26
let i = 65 + length + 6
return [...Array(length)]
.reduce(function (accumulator) {
return accumulator + String.fromCharCode(i++)
}, '')
}
| {
"content_hash": "acaae393142e1e7dd19c69d028125394",
"timestamp": "",
"source": "github",
"line_count": 13,
"max_line_length": 79,
"avg_line_length": 30.692307692307693,
"alnum_prop": 0.6516290726817042,
"repo_name": "kvz/phpjs",
"id": "a36b60226a6529a65f7a20da240227af599154e1",
"size": "399",
"binary": false,
"copies": "2",
"ref": "refs/heads/master",
"path": "src/python/string/ascii_lowercase.js",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "CSS",
"bytes": "90197"
},
{
"name": "HTML",
"bytes": "1848217"
},
{
"name": "JavaScript",
"bytes": "10460876"
},
{
"name": "Makefile",
"bytes": "2222"
},
{
"name": "PHP",
"bytes": "441330"
},
{
"name": "Ruby",
"bytes": "111087"
}
],
"symlink_target": ""
} |
require "test_helper"
class ShowcaseEchoRestServiceTest < PresenterTest
def presenter
service_presenter(:showcase, "Echo").rest
end
def test_name
assert_equal "Echo", presenter.name
end
def test_methods
refute_empty presenter.methods
presenter.methods.each { |ref| assert_kind_of Gapic::Presenters::MethodPresenter, ref }
# showcase does not have REST bindings for the `chat` method
# `expand` and `collect` are streaming methods and are currently not supported
exp_method_names = ["echo", "paged_expand", "wait", "block"]
assert_equal exp_method_names, presenter.methods.map(&:name)
end
def test_service_name_full
assert_equal "::Google::Showcase::V1beta1::Echo::Rest", presenter.service_name_full
end
def test_service_stub_name_full
assert_equal "::Google::Showcase::V1beta1::Echo::Rest::ServiceStub", presenter.service_stub_name_full
end
def test_operations_name
assert_equal "Operations", presenter.operations_name
end
def test_operations_name_full
assert_equal "::Google::Showcase::V1beta1::Echo::Rest::Operations", presenter.operations_name_full
end
def test_operations_file_path
assert_equal "google/showcase/v1beta1/echo/rest/operations.rb", presenter.operations_file_path
end
def test_operations_require
assert_equal "google/showcase/v1beta1/echo/rest/operations", presenter.operations_require
end
def test_test_client_file_path
assert_equal "google/showcase/v1beta1/echo_rest_test.rb", presenter.test_client_file_path
end
def test_lro?
assert presenter.lro?
end
def test_lro_service
assert_kind_of Gapic::Presenters::ServicePresenter, presenter.lro_service
end
end
| {
"content_hash": "cd5f3624e1a1c12399183c54f0b4db16",
"timestamp": "",
"source": "github",
"line_count": 56,
"max_line_length": 105,
"avg_line_length": 30.410714285714285,
"alnum_prop": 0.7369348209042865,
"repo_name": "googleapis/gapic-generator-ruby",
"id": "2e2d06874e5ca754ca4e404b65e1000af576bb18",
"size": "2310",
"binary": false,
"copies": "1",
"ref": "refs/heads/main",
"path": "gapic-generator/test/gapic/presenters/service/showcase_echo_rest_test.rb",
"mode": "33188",
"license": "apache-2.0",
"language": [
{
"name": "Dockerfile",
"bytes": "10623"
},
{
"name": "HTML",
"bytes": "136587"
},
{
"name": "Ruby",
"bytes": "5953831"
},
{
"name": "Shell",
"bytes": "36581"
},
{
"name": "Smarty",
"bytes": "2500"
},
{
"name": "Starlark",
"bytes": "91985"
}
],
"symlink_target": ""
} |
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<!--NewPage-->
<HTML>
<HEAD>
<TITLE>
Uses of Class org.apache.poi.poifs.storage.RawDataBlock (POI API Documentation)
</TITLE>
<LINK REL ="stylesheet" TYPE="text/css" HREF="../../../../../../stylesheet.css" TITLE="Style">
<SCRIPT type="text/javascript">
function windowTitle()
{
if (location.href.indexOf('is-external=true') == -1) {
parent.document.title="Uses of Class org.apache.poi.poifs.storage.RawDataBlock (POI API Documentation)";
}
}
</SCRIPT>
<NOSCRIPT>
</NOSCRIPT>
</HEAD>
<BODY BGCOLOR="white" onload="windowTitle();">
<HR>
<!-- ========= START OF TOP NAVBAR ======= -->
<A NAME="navbar_top"><!-- --></A>
<A HREF="#skip-navbar_top" title="Skip navigation links"></A>
<TABLE BORDER="0" WIDTH="100%" CELLPADDING="1" CELLSPACING="0" SUMMARY="">
<TR>
<TD COLSPAN=2 BGCOLOR="#EEEEFF" CLASS="NavBarCell1">
<A NAME="navbar_top_firstrow"><!-- --></A>
<TABLE BORDER="0" CELLPADDING="0" CELLSPACING="3" SUMMARY="">
<TR ALIGN="center" VALIGN="top">
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../../overview-summary.html"><FONT CLASS="NavBarFont1"><B>Overview</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../package-summary.html"><FONT CLASS="NavBarFont1"><B>Package</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../../org/apache/poi/poifs/storage/RawDataBlock.html" title="class in org.apache.poi.poifs.storage"><FONT CLASS="NavBarFont1"><B>Class</B></FONT></A> </TD>
<TD BGCOLOR="#FFFFFF" CLASS="NavBarCell1Rev"> <FONT CLASS="NavBarFont1Rev"><B>Use</B></FONT> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../package-tree.html"><FONT CLASS="NavBarFont1"><B>Tree</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../../deprecated-list.html"><FONT CLASS="NavBarFont1"><B>Deprecated</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../../index-all.html"><FONT CLASS="NavBarFont1"><B>Index</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../../help-doc.html"><FONT CLASS="NavBarFont1"><B>Help</B></FONT></A> </TD>
</TR>
</TABLE>
</TD>
<TD ALIGN="right" VALIGN="top" ROWSPAN=3><EM>
</EM>
</TD>
</TR>
<TR>
<TD BGCOLOR="white" CLASS="NavBarCell2"><FONT SIZE="-2">
PREV
NEXT</FONT></TD>
<TD BGCOLOR="white" CLASS="NavBarCell2"><FONT SIZE="-2">
<A HREF="../../../../../../index.html?org/apache/poi/poifs/storage/\class-useRawDataBlock.html" target="_top"><B>FRAMES</B></A>
<A HREF="RawDataBlock.html" target="_top"><B>NO FRAMES</B></A>
<SCRIPT type="text/javascript">
<!--
if(window==top) {
document.writeln('<A HREF="../../../../../../allclasses-noframe.html"><B>All Classes</B></A>');
}
//-->
</SCRIPT>
<NOSCRIPT>
<A HREF="../../../../../../allclasses-noframe.html"><B>All Classes</B></A>
</NOSCRIPT>
</FONT></TD>
</TR>
</TABLE>
<A NAME="skip-navbar_top"></A>
<!-- ========= END OF TOP NAVBAR ========= -->
<HR>
<CENTER>
<H2>
<B>Uses of Class<br>org.apache.poi.poifs.storage.RawDataBlock</B></H2>
</CENTER>
<TABLE BORDER="1" WIDTH="100%" CELLPADDING="3" CELLSPACING="0" SUMMARY="">
<TR BGCOLOR="#CCCCFF" CLASS="TableHeadingColor">
<TH ALIGN="left" COLSPAN="2"><FONT SIZE="+2">
Packages that use <A HREF="../../../../../../org/apache/poi/poifs/storage/RawDataBlock.html" title="class in org.apache.poi.poifs.storage">RawDataBlock</A></FONT></TH>
</TR>
<TR BGCOLOR="white" CLASS="TableRowColor">
<TD><A HREF="#org.apache.poi.poifs.filesystem"><B>org.apache.poi.poifs.filesystem</B></A></TD>
<TD>filesystem package maps OLE 2 Compound document files to a more familiar filesystem interface. </TD>
</TR>
<TR BGCOLOR="white" CLASS="TableRowColor">
<TD><A HREF="#org.apache.poi.poifs.storage"><B>org.apache.poi.poifs.storage</B></A></TD>
<TD>storage package contains low level binary structures for POIFS's implementation of the OLE 2
Compound Document Format. </TD>
</TR>
</TABLE>
<P>
<A NAME="org.apache.poi.poifs.filesystem"><!-- --></A>
<TABLE BORDER="1" WIDTH="100%" CELLPADDING="3" CELLSPACING="0" SUMMARY="">
<TR BGCOLOR="#CCCCFF" CLASS="TableHeadingColor">
<TH ALIGN="left" COLSPAN="2"><FONT SIZE="+2">
Uses of <A HREF="../../../../../../org/apache/poi/poifs/storage/RawDataBlock.html" title="class in org.apache.poi.poifs.storage">RawDataBlock</A> in <A HREF="../../../../../../org/apache/poi/poifs/filesystem/package-summary.html">org.apache.poi.poifs.filesystem</A></FONT></TH>
</TR>
</TABLE>
<P>
<TABLE BORDER="1" WIDTH="100%" CELLPADDING="3" CELLSPACING="0" SUMMARY="">
<TR BGCOLOR="#CCCCFF" CLASS="TableSubHeadingColor">
<TH ALIGN="left" COLSPAN="2">Constructors in <A HREF="../../../../../../org/apache/poi/poifs/filesystem/package-summary.html">org.apache.poi.poifs.filesystem</A> with parameters of type <A HREF="../../../../../../org/apache/poi/poifs/storage/RawDataBlock.html" title="class in org.apache.poi.poifs.storage">RawDataBlock</A></FONT></TH>
</TR>
<TR BGCOLOR="white" CLASS="TableRowColor">
<TD><CODE><B><A HREF="../../../../../../org/apache/poi/poifs/filesystem/OPOIFSDocument.html#OPOIFSDocument(java.lang.String, org.apache.poi.poifs.storage.RawDataBlock[], int)">OPOIFSDocument</A></B>(java.lang.String name,
<A HREF="../../../../../../org/apache/poi/poifs/storage/RawDataBlock.html" title="class in org.apache.poi.poifs.storage">RawDataBlock</A>[] blocks,
int length)</CODE>
<BR>
Constructor from large blocks</TD>
</TR>
</TABLE>
<P>
<A NAME="org.apache.poi.poifs.storage"><!-- --></A>
<TABLE BORDER="1" WIDTH="100%" CELLPADDING="3" CELLSPACING="0" SUMMARY="">
<TR BGCOLOR="#CCCCFF" CLASS="TableHeadingColor">
<TH ALIGN="left" COLSPAN="2"><FONT SIZE="+2">
Uses of <A HREF="../../../../../../org/apache/poi/poifs/storage/RawDataBlock.html" title="class in org.apache.poi.poifs.storage">RawDataBlock</A> in <A HREF="../../../../../../org/apache/poi/poifs/storage/package-summary.html">org.apache.poi.poifs.storage</A></FONT></TH>
</TR>
</TABLE>
<P>
<TABLE BORDER="1" WIDTH="100%" CELLPADDING="3" CELLSPACING="0" SUMMARY="">
<TR BGCOLOR="#CCCCFF" CLASS="TableSubHeadingColor">
<TH ALIGN="left" COLSPAN="2">Constructors in <A HREF="../../../../../../org/apache/poi/poifs/storage/package-summary.html">org.apache.poi.poifs.storage</A> with parameters of type <A HREF="../../../../../../org/apache/poi/poifs/storage/RawDataBlock.html" title="class in org.apache.poi.poifs.storage">RawDataBlock</A></FONT></TH>
</TR>
<TR BGCOLOR="white" CLASS="TableRowColor">
<TD><CODE><B><A HREF="../../../../../../org/apache/poi/poifs/storage/DocumentBlock.html#DocumentBlock(org.apache.poi.poifs.storage.RawDataBlock)">DocumentBlock</A></B>(<A HREF="../../../../../../org/apache/poi/poifs/storage/RawDataBlock.html" title="class in org.apache.poi.poifs.storage">RawDataBlock</A> block)</CODE>
<BR>
create a document block from a raw data block</TD>
</TR>
</TABLE>
<P>
<HR>
<!-- ======= START OF BOTTOM NAVBAR ====== -->
<A NAME="navbar_bottom"><!-- --></A>
<A HREF="#skip-navbar_bottom" title="Skip navigation links"></A>
<TABLE BORDER="0" WIDTH="100%" CELLPADDING="1" CELLSPACING="0" SUMMARY="">
<TR>
<TD COLSPAN=2 BGCOLOR="#EEEEFF" CLASS="NavBarCell1">
<A NAME="navbar_bottom_firstrow"><!-- --></A>
<TABLE BORDER="0" CELLPADDING="0" CELLSPACING="3" SUMMARY="">
<TR ALIGN="center" VALIGN="top">
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../../overview-summary.html"><FONT CLASS="NavBarFont1"><B>Overview</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../package-summary.html"><FONT CLASS="NavBarFont1"><B>Package</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../../org/apache/poi/poifs/storage/RawDataBlock.html" title="class in org.apache.poi.poifs.storage"><FONT CLASS="NavBarFont1"><B>Class</B></FONT></A> </TD>
<TD BGCOLOR="#FFFFFF" CLASS="NavBarCell1Rev"> <FONT CLASS="NavBarFont1Rev"><B>Use</B></FONT> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../package-tree.html"><FONT CLASS="NavBarFont1"><B>Tree</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../../deprecated-list.html"><FONT CLASS="NavBarFont1"><B>Deprecated</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../../index-all.html"><FONT CLASS="NavBarFont1"><B>Index</B></FONT></A> </TD>
<TD BGCOLOR="#EEEEFF" CLASS="NavBarCell1"> <A HREF="../../../../../../help-doc.html"><FONT CLASS="NavBarFont1"><B>Help</B></FONT></A> </TD>
</TR>
</TABLE>
</TD>
<TD ALIGN="right" VALIGN="top" ROWSPAN=3><EM>
</EM>
</TD>
</TR>
<TR>
<TD BGCOLOR="white" CLASS="NavBarCell2"><FONT SIZE="-2">
PREV
NEXT</FONT></TD>
<TD BGCOLOR="white" CLASS="NavBarCell2"><FONT SIZE="-2">
<A HREF="../../../../../../index.html?org/apache/poi/poifs/storage/\class-useRawDataBlock.html" target="_top"><B>FRAMES</B></A>
<A HREF="RawDataBlock.html" target="_top"><B>NO FRAMES</B></A>
<SCRIPT type="text/javascript">
<!--
if(window==top) {
document.writeln('<A HREF="../../../../../../allclasses-noframe.html"><B>All Classes</B></A>');
}
//-->
</SCRIPT>
<NOSCRIPT>
<A HREF="../../../../../../allclasses-noframe.html"><B>All Classes</B></A>
</NOSCRIPT>
</FONT></TD>
</TR>
</TABLE>
<A NAME="skip-navbar_bottom"></A>
<!-- ======== END OF BOTTOM NAVBAR ======= -->
<HR>
<i>Copyright 2015 The Apache Software Foundation or
its licensors, as applicable.</i>
</BODY>
</HTML>
| {
"content_hash": "d310ab72f8f7b613ab4fd96ce502cda5",
"timestamp": "",
"source": "github",
"line_count": 209,
"max_line_length": 335,
"avg_line_length": 48.67942583732057,
"alnum_prop": 0.6226656182425792,
"repo_name": "xiwan/xlsEditor",
"id": "e6e94b51a89bd6874c0d449d9ab54263868e9ca5",
"size": "10174",
"binary": false,
"copies": "2",
"ref": "refs/heads/master",
"path": "lib/poi-3.13/docs/apidocs/org/apache/poi/poifs/storage/class-use/RawDataBlock.html",
"mode": "33188",
"license": "mit",
"language": [
{
"name": "CSS",
"bytes": "42792"
},
{
"name": "Emacs Lisp",
"bytes": "1320"
},
{
"name": "HTML",
"bytes": "107233820"
},
{
"name": "Java",
"bytes": "2866828"
},
{
"name": "Lex",
"bytes": "9342"
}
],
"symlink_target": ""
} |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.