code
stringlengths 2
1.05M
| repo_name
stringlengths 5
104
| path
stringlengths 4
251
| language
stringclasses 1
value | license
stringclasses 15
values | size
int32 2
1.05M
|
---|---|---|---|---|---|
#!/pyenv/bin/python
import sys, json, numpy as np
from sklearn.preprocessing import LabelEncoder
from sklearn.cluster import KMeans
x = []
f = open('cluster_input.txt')
lines = f.read().split('\n')
#for line in sys.stdin:
for idx, line in enumerate(lines[:-1]):
print idx
tokens = line.strip().split('\t')
points = []
for point in json.loads(tokens[9]):
points.append(point['x'])
points.append(point['y'])
while len(points) < 20:
points.append(-999.25)
points.append(-999.25)
#mnemonic, desription, uom, average, median, stddev, variance, max, min, range, histogram points
if len(tokens) == 11: description = tokens[10]
else: description = ''
x.append((tokens[0], description, tokens[1], \
float(tokens[2]), float(tokens[3]), float(tokens[4]), float(tokens[5]), float(tokens[6]), float(tokens[7]), float(tokens[8]), \
points[0], points[1], points[2], points[3], points[4], points[5], points[6], points[7], points[8], points[9], points[10], points[11], points[12], \
points[13], points[14], points[15], points[16], points[17], points[18], points[19]))
X = np.asarray(x)
print 'Label encoding mnemonic'
X[:,0] = LabelEncoder().fit_transform(X[:,0]) #mnemonic
print 'Label encoding description'
X[:,1] = LabelEncoder().fit_transform(X[:,1]) #description
print 'Label encoding uom'
X[:,2] = LabelEncoder().fit_transform(X[:,2]) #uom
#f = open('cluster_input-encoded.txt')
print 'Beginning clustering..'
#clusters = KMeans(init='k-means++', n_clusters=10, n_init=10, name='k-means++', data=X)
print 'Finished!'
| randerzander/wellbook | etl/hive/cluster.py | Python | apache-2.0 | 1,557 |
from Urutu import *
import numpy as np
@Urutu("CL")
def divmul(a, b, c, d):
Tx, Ty, Tz = 100, 1, 1
Bx, By, Bz = 1, 1, 1
c[tx] = a[tx] / b[tx]
d[tx] = a[tx] * b[tx]
return c, d
@Urutu("CU")
def addsub(a, b, e, f):
Tx, Ty, Tz = 100, 1, 1
Bx, By, Bz = 1, 1, 1
e[tx] = a[tx] + b[tx]
f[tx] = a[tx] - b[tx]
return e, f
a = np.random.randint(10, size = 100)
b = np.random.randint(10, size = 100)
c = np.array(a, dtype = 'f')
d = np.empty_like(a)
e = np.empty_like(a)
f = np.empty_like(a)
print "The Array A is: \n",a
print "The Array B is: \n",b
print "Running on OpenCL.. \n",divmul(a, b, c, d)
print "Running on CUDA.. \n",addsub(a, b, e, f)
| adityaatluri/Urutu | test/test_simple.py | Python | apache-2.0 | 651 |
# -*- encoding: utf-8 -*-
#
# Copyright © 2012 New Dream Network, LLC (DreamHost)
#
# Author: Doug Hellmann <[email protected]>
#
# Licensed under the Apache License, Version 2.0 (the "License"); you may
# not use this file except in compliance with the License. You may obtain
# a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
# License for the specific language governing permissions and limitations
# under the License.
"""Blueprint for version 1 of API.
"""
#############################################################################
#############################################################################
#
# NOTE(dhellmann): The V1 API is being deprecated during Havana, and
# as such it is feature-frozen. Only make bug-fix changes in this file.
#
#############################################################################
#############################################################################
# [ ] / -- information about this version of the API
#
# [ ] /extensions -- list of available extensions
# [ ] /extensions/<extension> -- details about a specific extension
#
# [ ] /sources -- list of known sources (where do we get this?)
# [ ] /sources/components -- list of components which provide metering
# data (where do we get this)?
#
# [x] /projects/<project>/resources -- list of resource ids
# [x] /resources -- list of resource ids
# [x] /sources/<source>/resources -- list of resource ids
# [x] /users/<user>/resources -- list of resource ids
#
# [x] /users -- list of user ids
# [x] /sources/<source>/users -- list of user ids
#
# [x] /projects -- list of project ids
# [x] /sources/<source>/projects -- list of project ids
#
# [ ] /resources/<resource> -- metadata
#
# [x] /meters -- list of meters
# [x] /projects/<project>/meters -- list of meters reporting for parent obj
# [x] /resources/<resource>/meters -- list of meters reporting for parent obj
# [x] /sources/<source>/meters -- list of meters reporting for parent obj
# [x] /users/<user>/meters -- list of meters reporting for parent obj
#
# [x] /projects/<project>/meters/<meter> -- samples
# [x] /resources/<resource>/meters/<meter> -- samples
# [x] /sources/<source>/meters/<meter> -- samples
# [x] /users/<user>/meters/<meter> -- samples
#
# [ ] /projects/<project>/meters/<meter>/duration -- total time for selected
# meter
# [x] /resources/<resource>/meters/<meter>/duration -- total time for selected
# meter
# [ ] /sources/<source>/meters/<meter>/duration -- total time for selected
# meter
# [ ] /users/<user>/meters/<meter>/duration -- total time for selected meter
#
# [ ] /projects/<project>/meters/<meter>/volume -- total or max volume for
# selected meter
# [x] /projects/<project>/meters/<meter>/volume/max -- max volume for
# selected meter
# [x] /projects/<project>/meters/<meter>/volume/sum -- total volume for
# selected meter
# [ ] /resources/<resource>/meters/<meter>/volume -- total or max volume for
# selected meter
# [x] /resources/<resource>/meters/<meter>/volume/max -- max volume for
# selected meter
# [x] /resources/<resource>/meters/<meter>/volume/sum -- total volume for
# selected meter
# [ ] /sources/<source>/meters/<meter>/volume -- total or max volume for
# selected meter
# [ ] /users/<user>/meters/<meter>/volume -- total or max volume for selected
# meter
import datetime
import flask
from ceilometer.openstack.common.gettextutils import _
from ceilometer.openstack.common import log
from ceilometer.openstack.common import timeutils
from ceilometer import storage
from ceilometer.api import acl
LOG = log.getLogger(__name__)
blueprint = flask.Blueprint('v1', __name__,
template_folder='templates',
static_folder='static')
def request_wants_html():
best = flask.request.accept_mimetypes \
.best_match(['application/json', 'text/html'])
return best == 'text/html' and \
flask.request.accept_mimetypes[best] > \
flask.request.accept_mimetypes['application/json']
def _get_metaquery(args):
return dict((k, v)
for (k, v) in args.iteritems()
if k.startswith('metadata.'))
def check_authorized_project(project):
authorized_project = acl.get_limited_to_project(flask.request.headers)
if authorized_project and authorized_project != project:
flask.abort(404)
## APIs for working with meters.
@blueprint.route('/meters')
def list_meters_all():
"""Return a list of meters.
:param metadata.<key>: match on the metadata within the resource.
(optional)
"""
rq = flask.request
meters = rq.storage_conn.get_meters(
project=acl.get_limited_to_project(rq.headers),
metaquery=_get_metaquery(rq.args))
return flask.jsonify(meters=[m.as_dict() for m in meters])
@blueprint.route('/resources/<resource>/meters')
def list_meters_by_resource(resource):
"""Return a list of meters by resource.
:param resource: The ID of the resource.
:param metadata.<key>: match on the metadata within the resource.
(optional)
"""
rq = flask.request
meters = rq.storage_conn.get_meters(
resource=resource,
project=acl.get_limited_to_project(rq.headers),
metaquery=_get_metaquery(rq.args))
return flask.jsonify(meters=[m.as_dict() for m in meters])
@blueprint.route('/users/<user>/meters')
def list_meters_by_user(user):
"""Return a list of meters by user.
:param user: The ID of the owning user.
:param metadata.<key>: match on the metadata within the resource.
(optional)
"""
rq = flask.request
meters = rq.storage_conn.get_meters(
user=user,
project=acl.get_limited_to_project(rq.headers),
metaquery=_get_metaquery(rq.args))
return flask.jsonify(meters=[m.as_dict() for m in meters])
@blueprint.route('/projects/<project>/meters')
def list_meters_by_project(project):
"""Return a list of meters by project.
:param project: The ID of the owning project.
:param metadata.<key>: match on the metadata within the resource.
(optional)
"""
check_authorized_project(project)
rq = flask.request
meters = rq.storage_conn.get_meters(
project=project,
metaquery=_get_metaquery(rq.args))
return flask.jsonify(meters=[m.as_dict() for m in meters])
@blueprint.route('/sources/<source>/meters')
def list_meters_by_source(source):
"""Return a list of meters by source.
:param source: The ID of the owning source.
:param metadata.<key>: match on the metadata within the resource.
(optional)
"""
rq = flask.request
meters = rq.storage_conn.get_meters(
source=source,
project=acl.get_limited_to_project(rq.headers),
metaquery=_get_metaquery(rq.args))
return flask.jsonify(meters=[m.as_dict() for m in meters])
## APIs for working with resources.
def _list_resources(source=None, user=None, project=None):
"""Return a list of resource identifiers.
"""
rq = flask.request
q_ts = _get_query_timestamps(rq.args)
resources = rq.storage_conn.get_resources(
source=source,
user=user,
project=project,
start_timestamp=q_ts['start_timestamp'],
end_timestamp=q_ts['end_timestamp'],
metaquery=_get_metaquery(rq.args),
)
return flask.jsonify(resources=[r.as_dict() for r in resources])
@blueprint.route('/projects/<project>/resources')
def list_resources_by_project(project):
"""Return a list of resources owned by the project.
:param project: The ID of the owning project.
:param start_timestamp: Limits resources by last update time >= this value.
(optional)
:type start_timestamp: ISO date in UTC
:param end_timestamp: Limits resources by last update time < this value.
(optional)
:type end_timestamp: ISO date in UTC
:param metadata.<key>: match on the metadata within the resource.
(optional)
"""
check_authorized_project(project)
return _list_resources(project=project)
@blueprint.route('/resources')
def list_all_resources():
"""Return a list of all known resources.
:param start_timestamp: Limits resources by last update time >= this value.
(optional)
:type start_timestamp: ISO date in UTC
:param end_timestamp: Limits resources by last update time < this value.
(optional)
:type end_timestamp: ISO date in UTC
:param metadata.<key>: match on the metadata within the resource.
(optional)
"""
return _list_resources(
project=acl.get_limited_to_project(flask.request.headers))
@blueprint.route('/sources/<source>')
def get_source(source):
"""Return a source details.
:param source: The ID of the reporting source.
"""
return flask.jsonify(flask.request.sources.get(source, {}))
@blueprint.route('/sources/<source>/resources')
def list_resources_by_source(source):
"""Return a list of resources for which a source is reporting
data.
:param source: The ID of the reporting source.
:param start_timestamp: Limits resources by last update time >= this value.
(optional)
:type start_timestamp: ISO date in UTC
:param end_timestamp: Limits resources by last update time < this value.
(optional)
:type end_timestamp: ISO date in UTC
:param metadata.<key>: match on the metadata within the resource.
(optional)
"""
return _list_resources(
source=source,
project=acl.get_limited_to_project(flask.request.headers),
)
@blueprint.route('/users/<user>/resources')
def list_resources_by_user(user):
"""Return a list of resources owned by the user.
:param user: The ID of the owning user.
:param start_timestamp: Limits resources by last update time >= this value.
(optional)
:type start_timestamp: ISO date in UTC
:param end_timestamp: Limits resources by last update time < this value.
(optional)
:type end_timestamp: ISO date in UTC
:param metadata.<key>: match on the metadata within the resource.
(optional)
"""
return _list_resources(
user=user,
project=acl.get_limited_to_project(flask.request.headers),
)
## APIs for working with users.
def _list_users(source=None):
"""Return a list of user names.
"""
# TODO(jd) it might be better to return the real list of users that are
# belonging to the project, but that's not provided by the storage
# drivers for now
if acl.get_limited_to_project(flask.request.headers):
users = [flask.request.headers.get('X-User-id')]
else:
users = flask.request.storage_conn.get_users(source=source)
return flask.jsonify(users=list(users))
@blueprint.route('/users')
def list_all_users():
"""Return a list of all known user names.
"""
return _list_users()
@blueprint.route('/sources/<source>/users')
def list_users_by_source(source):
"""Return a list of the users for which the source is reporting
data.
:param source: The ID of the source.
"""
return _list_users(source=source)
## APIs for working with projects.
def _list_projects(source=None):
"""Return a list of project names.
"""
project = acl.get_limited_to_project(flask.request.headers)
if project:
if source:
if project in flask.request.storage_conn.get_projects(
source=source):
projects = [project]
else:
projects = []
else:
projects = [project]
else:
projects = flask.request.storage_conn.get_projects(source=source)
return flask.jsonify(projects=list(projects))
@blueprint.route('/projects')
def list_all_projects():
"""Return a list of all known project names.
"""
return _list_projects()
@blueprint.route('/sources/<source>/projects')
def list_projects_by_source(source):
"""Return a list project names for which the source is reporting
data.
:param source: The ID of the source.
"""
return _list_projects(source=source)
## APIs for working with samples.
def _list_samples(meter,
project=None,
resource=None,
source=None,
user=None):
"""Return a list of raw samples.
Note: the API talks about "events" these are equivalent to samples.
but we still need to return the samples within the "events" dict
to maintain API compatibilty.
"""
q_ts = _get_query_timestamps(flask.request.args)
f = storage.SampleFilter(
user=user,
project=project,
source=source,
meter=meter,
resource=resource,
start=q_ts['start_timestamp'],
end=q_ts['end_timestamp'],
metaquery=_get_metaquery(flask.request.args),
)
samples = flask.request.storage_conn.get_samples(f)
jsonified = flask.jsonify(events=[s.as_dict() for s in samples])
if request_wants_html():
return flask.templating.render_template('list_event.html',
user=user,
project=project,
source=source,
meter=meter,
resource=resource,
events=jsonified)
return jsonified
@blueprint.route('/projects/<project>/meters/<meter>')
def list_samples_by_project(project, meter):
"""Return a list of raw samples for the project.
:param project: The ID of the project.
:param meter: The name of the meter.
:param start_timestamp: Limits samples by timestamp >= this value.
(optional)
:type start_timestamp: ISO date in UTC
:param end_timestamp: Limits samples by timestamp < this value.
(optional)
:type end_timestamp: ISO date in UTC
"""
check_authorized_project(project)
return _list_samples(project=project,
meter=meter,
)
@blueprint.route('/resources/<resource>/meters/<meter>')
def list_samples_by_resource(resource, meter):
"""Return a list of raw samples for the resource.
:param resource: The ID of the resource.
:param meter: The name of the meter.
:param start_timestamp: Limits samples by timestamp >= this value.
(optional)
:type start_timestamp: ISO date in UTC
:param end_timestamp: Limits samples by timestamp < this value.
(optional)
:type end_timestamp: ISO date in UTC
"""
return _list_samples(
resource=resource,
meter=meter,
project=acl.get_limited_to_project(flask.request.headers),
)
@blueprint.route('/sources/<source>/meters/<meter>')
def list_samples_by_source(source, meter):
"""Return a list of raw samples for the source.
:param source: The ID of the reporting source.
:param meter: The name of the meter.
:param start_timestamp: Limits samples by timestamp >= this value.
(optional)
:type start_timestamp: ISO date in UTC
:param end_timestamp: Limits samples by timestamp < this value.
(optional)
:type end_timestamp: ISO date in UTC
"""
return _list_samples(
source=source,
meter=meter,
project=acl.get_limited_to_project(flask.request.headers),
)
@blueprint.route('/users/<user>/meters/<meter>')
def list_samples_by_user(user, meter):
"""Return a list of raw samples for the user.
:param user: The ID of the user.
:param meter: The name of the meter.
:param start_timestamp: Limits samples by timestamp >= this value.
(optional)
:type start_timestamp: ISO date in UTC
:param end_timestamp: Limits samples by timestamp < this value.
(optional)
:type end_timestamp: ISO date in UTC
"""
return _list_samples(
user=user,
meter=meter,
project=acl.get_limited_to_project(flask.request.headers),
)
## APIs for working with meter calculations.
def _get_query_timestamps(args={}):
# Determine the desired range, if any, from the
# GET arguments. Set up the query range using
# the specified offset.
# [query_start ... start_timestamp ... end_timestamp ... query_end]
search_offset = int(args.get('search_offset', 0))
start_timestamp = args.get('start_timestamp')
if start_timestamp:
start_timestamp = timeutils.parse_isotime(start_timestamp)
start_timestamp = start_timestamp.replace(tzinfo=None)
query_start = (start_timestamp -
datetime.timedelta(minutes=search_offset))
else:
query_start = None
end_timestamp = args.get('end_timestamp')
if end_timestamp:
end_timestamp = timeutils.parse_isotime(end_timestamp)
end_timestamp = end_timestamp.replace(tzinfo=None)
query_end = end_timestamp + datetime.timedelta(minutes=search_offset)
else:
query_end = None
return dict(query_start=query_start,
query_end=query_end,
start_timestamp=start_timestamp,
end_timestamp=end_timestamp,
search_offset=search_offset,
)
@blueprint.route('/resources/<resource>/meters/<meter>/duration')
def compute_duration_by_resource(resource, meter):
"""Return the earliest timestamp, last timestamp,
and duration for the resource and meter.
:param resource: The ID of the resource.
:param meter: The name of the meter.
:param start_timestamp: ISO-formatted string of the
earliest timestamp to return.
:param end_timestamp: ISO-formatted string of the
latest timestamp to return.
:param search_offset: Number of minutes before
and after start and end timestamps to query.
"""
q_ts = _get_query_timestamps(flask.request.args)
start_timestamp = q_ts['start_timestamp']
end_timestamp = q_ts['end_timestamp']
# Query the database for the interval of timestamps
# within the desired range.
f = storage.SampleFilter(
meter=meter,
project=acl.get_limited_to_project(flask.request.headers),
resource=resource,
start=q_ts['query_start'],
end=q_ts['query_end'],
)
stats = flask.request.storage_conn.get_meter_statistics(f)
min_ts, max_ts = stats.duration_start, stats.duration_end
# "Clamp" the timestamps we return to the original time
# range, excluding the offset.
LOG.debug(_('start_timestamp %(start_timestamp)s, '
'end_timestamp %(end_timestamp)s, '
'min_ts %(min_ts)s, '
'max_ts %(max_ts)s') % (
{'start_timestamp': start_timestamp,
'end_timestamp': end_timestamp,
'min_ts': min_ts,
'max_ts': max_ts}))
if start_timestamp and min_ts and min_ts < start_timestamp:
min_ts = start_timestamp
LOG.debug(_('clamping min timestamp to range'))
if end_timestamp and max_ts and max_ts > end_timestamp:
max_ts = end_timestamp
LOG.debug(_('clamping max timestamp to range'))
# If we got valid timestamps back, compute a duration in minutes.
#
# If the min > max after clamping then we know the
# timestamps on the samples fell outside of the time
# range we care about for the query, so treat them as
# "invalid."
#
# If the timestamps are invalid, return None as a
# sentinal indicating that there is something "funny"
# about the range.
if min_ts and max_ts and (min_ts <= max_ts):
duration = timeutils.delta_seconds(min_ts, max_ts)
else:
min_ts = max_ts = duration = None
return flask.jsonify(start_timestamp=min_ts,
end_timestamp=max_ts,
duration=duration,
)
def _get_statistics(stats_type, meter=None, resource=None, project=None):
q_ts = _get_query_timestamps(flask.request.args)
f = storage.SampleFilter(
meter=meter,
project=project,
resource=resource,
start=q_ts['query_start'],
end=q_ts['query_end'],
)
# TODO(sberler): do we want to return an error if the resource
# does not exist?
results = list(flask.request.storage_conn.get_meter_statistics(f))
value = None
if results:
value = getattr(results[0], stats_type) # there should only be one!
return flask.jsonify(volume=value)
@blueprint.route('/resources/<resource>/meters/<meter>/volume/max')
def compute_max_resource_volume(resource, meter):
"""Return the max volume for a meter.
:param resource: The ID of the resource.
:param meter: The name of the meter.
:param start_timestamp: ISO-formatted string of the
earliest time to include in the calculation.
:param end_timestamp: ISO-formatted string of the
latest time to include in the calculation.
:param search_offset: Number of minutes before and
after start and end timestamps to query.
"""
return _get_statistics(
'max',
meter=meter,
resource=resource,
project=acl.get_limited_to_project(flask.request.headers),
)
@blueprint.route('/resources/<resource>/meters/<meter>/volume/sum')
def compute_resource_volume_sum(resource, meter):
"""Return the sum of samples for a meter.
:param resource: The ID of the resource.
:param meter: The name of the meter.
:param start_timestamp: ISO-formatted string of the
earliest time to include in the calculation.
:param end_timestamp: ISO-formatted string of the
latest time to include in the calculation.
:param search_offset: Number of minutes before and
after start and end timestamps to query.
"""
return _get_statistics(
'sum',
meter=meter,
resource=resource,
project=acl.get_limited_to_project(flask.request.headers),
)
@blueprint.route('/projects/<project>/meters/<meter>/volume/max')
def compute_project_volume_max(project, meter):
"""Return the max volume for a meter.
:param project: The ID of the project.
:param meter: The name of the meter.
:param start_timestamp: ISO-formatted string of the
earliest time to include in the calculation.
:param end_timestamp: ISO-formatted string of the
latest time to include in the calculation.
:param search_offset: Number of minutes before and
after start and end timestamps to query.
"""
check_authorized_project(project)
return _get_statistics('max', project=project, meter=meter)
@blueprint.route('/projects/<project>/meters/<meter>/volume/sum')
def compute_project_volume_sum(project, meter):
"""Return the total volume for a meter.
:param project: The ID of the project.
:param meter: The name of the meter.
:param start_timestamp: ISO-formatted string of the
earliest time to include in the calculation.
:param end_timestamp: ISO-formatted string of the
latest time to include in the calculation.
:param search_offset: Number of minutes before and
after start and end timestamps to query.
"""
check_authorized_project(project)
return _get_statistics(
'sum',
meter=meter,
project=project,
)
| tanglei528/ceilometer | ceilometer/api/v1/blueprint.py | Python | apache-2.0 | 24,451 |
# -*- coding: utf-8 -*-
from AppiumLibrary import utils
from robot.api import logger
class ElementFinder(object):
def __init__(self):
self._strategies = {
'identifier': self._find_by_identifier,
'id': self._find_by_id,
'name': self._find_by_name,
'xpath': self._find_by_xpath,
'class': self._find_by_class_name,
'accessibility_id': self._find_element_by_accessibility_id,
'android': self._find_by_android,
'ios': self._find_by_ios,
'css': self._find_by_css_selector,
'jquery': self._find_by_sizzle_selector,
'nsp': self._find_by_nsp,
'chain': self._find_by_chain,
'default': self._find_by_default
}
def find(self, browser, locator, tag=None):
assert browser is not None
assert locator is not None and len(locator) > 0
(prefix, criteria) = self._parse_locator(locator)
prefix = 'default' if prefix is None else prefix
strategy = self._strategies.get(prefix)
if strategy is None:
raise ValueError("Element locator with prefix '" + prefix + "' is not supported")
(tag, constraints) = self._get_tag_and_constraints(tag)
return strategy(browser, criteria, tag, constraints)
# Strategy routines, private
def _find_by_identifier(self, browser, criteria, tag, constraints):
elements = self._normalize_result(browser.find_elements_by_id(criteria))
elements.extend(self._normalize_result(browser.find_elements_by_name(criteria)))
return self._filter_elements(elements, tag, constraints)
def _find_by_id(self, browser, criteria, tag, constraints):
return self._filter_elements(
browser.find_elements_by_id(criteria),
tag, constraints)
def _find_by_name(self, browser, criteria, tag, constraints):
return self._filter_elements(
browser.find_elements_by_name(criteria),
tag, constraints)
def _find_by_xpath(self, browser, criteria, tag, constraints):
return self._filter_elements(
browser.find_elements_by_xpath(criteria),
tag, constraints)
def _find_by_dom(self, browser, criteria, tag, constraints):
result = browser.execute_script("return %s;" % criteria)
if result is None:
return []
if not isinstance(result, list):
result = [result]
return self._filter_elements(result, tag, constraints)
def _find_by_sizzle_selector(self, browser, criteria, tag, constraints):
js = "return jQuery('%s').get();" % criteria.replace("'", "\\'")
return self._filter_elements(
browser.execute_script(js),
tag, constraints)
def _find_by_link_text(self, browser, criteria, tag, constraints):
return self._filter_elements(
browser.find_elements_by_link_text(criteria),
tag, constraints)
def _find_by_css_selector(self, browser, criteria, tag, constraints):
return self._filter_elements(
browser.find_elements_by_css_selector(criteria),
tag, constraints)
def _find_by_tag_name(self, browser, criteria, tag, constraints):
return self._filter_elements(
browser.find_elements_by_tag_name(criteria),
tag, constraints)
def _find_by_class_name(self, browser, criteria, tag, constraints):
return self._filter_elements(
browser.find_elements_by_class_name(criteria),
tag, constraints)
def _find_element_by_accessibility_id(self, browser, criteria, tag, constraints):
elements = browser.find_elements_by_accessibility_id(criteria)
return elements
def _find_by_android(self, browser, criteria, tag, constraints):
"""Find element matches by UI Automator."""
return self._filter_elements(
browser.find_elements_by_android_uiautomator(criteria),
tag, constraints)
def _find_by_ios(self, browser, criteria, tag, constraints):
"""Find element matches by UI Automation."""
return self._filter_elements(
browser.find_elements_by_ios_uiautomation(criteria),
tag, constraints)
def _find_by_nsp(self, browser, criteria, tag, constraints):
"""Find element matches by iOSNsPredicateString."""
return self._filter_elements(
browser.find_elements_by_ios_predicate(criteria),
tag, constraints)
def _find_by_chain(self, browser, criteria, tag, constraints):
"""Find element matches by iOSChainString."""
return self._filter_elements(
browser.find_elements_by_ios_class_chain(criteria),
tag, constraints)
def _find_by_default(self, browser, criteria, tag, constraints):
if criteria.startswith('//'):
return self._find_by_xpath(browser, criteria, tag, constraints)
# Used `id` instead of _find_by_key_attrs since iOS and Android internal `id` alternatives are
# different and inside appium python client. Need to expose these and improve in order to make
# _find_by_key_attrs useful.
return self._find_by_id(browser, criteria, tag, constraints)
# TODO: Not in use after conversion from Selenium2Library need to make more use of multiple auto selector strategy
def _find_by_key_attrs(self, browser, criteria, tag, constraints):
key_attrs = self._key_attrs.get(None)
if tag is not None:
key_attrs = self._key_attrs.get(tag, key_attrs)
xpath_criteria = utils.escape_xpath_value(criteria)
xpath_tag = tag if tag is not None else '*'
xpath_constraints = ["@%s='%s'" % (name, constraints[name]) for name in constraints]
xpath_searchers = ["%s=%s" % (attr, xpath_criteria) for attr in key_attrs]
xpath_searchers.extend(
self._get_attrs_with_url(key_attrs, criteria, browser))
xpath = "//%s[%s(%s)]" % (
xpath_tag,
' and '.join(xpath_constraints) + ' and ' if len(xpath_constraints) > 0 else '',
' or '.join(xpath_searchers))
return self._normalize_result(browser.find_elements_by_xpath(xpath))
# Private
_key_attrs = {
None: ['@id', '@name'],
'a': ['@id', '@name', '@href', 'normalize-space(descendant-or-self::text())'],
'img': ['@id', '@name', '@src', '@alt'],
'input': ['@id', '@name', '@value', '@src'],
'button': ['@id', '@name', '@value', 'normalize-space(descendant-or-self::text())']
}
def _get_tag_and_constraints(self, tag):
if tag is None:
return None, {}
tag = tag.lower()
constraints = {}
if tag == 'link':
tag = 'a'
elif tag == 'image':
tag = 'img'
elif tag == 'list':
tag = 'select'
elif tag == 'radio button':
tag = 'input'
constraints['type'] = 'radio'
elif tag == 'checkbox':
tag = 'input'
constraints['type'] = 'checkbox'
elif tag == 'text field':
tag = 'input'
constraints['type'] = 'text'
elif tag == 'file upload':
tag = 'input'
constraints['type'] = 'file'
return tag, constraints
def _element_matches(self, element, tag, constraints):
if not element.tag_name.lower() == tag:
return False
for name in constraints:
if not element.get_attribute(name) == constraints[name]:
return False
return True
def _filter_elements(self, elements, tag, constraints):
elements = self._normalize_result(elements)
if tag is None:
return elements
return filter(
lambda element: self._element_matches(element, tag, constraints),
elements)
def _get_attrs_with_url(self, key_attrs, criteria, browser):
attrs = []
url = None
xpath_url = None
for attr in ['@src', '@href']:
if attr in key_attrs:
if url is None or xpath_url is None:
url = self._get_base_url(browser) + "/" + criteria
xpath_url = utils.escape_xpath_value(url)
attrs.append("%s=%s" % (attr, xpath_url))
return attrs
def _get_base_url(self, browser):
url = browser.get_current_url()
if '/' in url:
url = '/'.join(url.split('/')[:-1])
return url
def _parse_locator(self, locator):
prefix = None
criteria = locator
if not locator.startswith('//'):
locator_parts = locator.partition('=')
if len(locator_parts[1]) > 0:
prefix = locator_parts[0].strip().lower()
criteria = locator_parts[2].strip()
return (prefix, criteria)
def _normalize_result(self, elements):
if not isinstance(elements, list):
logger.debug("WebDriver find returned %s" % elements)
return []
return elements
| serhatbolsu/robotframework-appiumlibrary | AppiumLibrary/locators/elementfinder.py | Python | apache-2.0 | 9,396 |
# This Source Code Form is subject to the terms of the Mozilla Public
# License, v. 2.0. If a copy of the MPL was not distributed with this
# file, You can obtain one at http://mozilla.org/MPL/2.0/.
import os
from marionette_test import MarionetteTestCase
from marionette import HTMLElement
from errors import NoSuchElementException, JavascriptException, MarionetteException, ScriptTimeoutException
class TestTimeouts(MarionetteTestCase):
def test_pagetimeout_notdefinetimeout_pass(self):
test_html = self.marionette.absolute_url("test.html")
self.marionette.navigate(test_html)
def test_pagetimeout_fail(self):
self.marionette.timeouts("page load", 0)
test_html = self.marionette.absolute_url("test.html")
self.assertRaises(MarionetteException, self.marionette.navigate, test_html)
def test_pagetimeout_pass(self):
self.marionette.timeouts("page load", 60000)
test_html = self.marionette.absolute_url("test.html")
self.marionette.navigate(test_html)
def test_searchtimeout_notfound_settimeout(self):
test_html = self.marionette.absolute_url("test.html")
self.marionette.navigate(test_html)
self.marionette.timeouts("implicit", 1000)
self.assertRaises(NoSuchElementException, self.marionette.find_element, "id", "I'm not on the page")
self.marionette.timeouts("implicit", 0)
self.assertRaises(NoSuchElementException, self.marionette.find_element, "id", "I'm not on the page")
def test_searchtimeout_found_settimeout(self):
test_html = self.marionette.absolute_url("test.html")
self.marionette.navigate(test_html)
self.marionette.timeouts("implicit", 4000)
self.assertEqual(HTMLElement, type(self.marionette.find_element("id", "newDiv")))
def test_searchtimeout_found(self):
test_html = self.marionette.absolute_url("test.html")
self.marionette.navigate(test_html)
self.assertRaises(NoSuchElementException, self.marionette.find_element, "id", "newDiv")
def test_execute_async_timeout_settimeout(self):
test_html = self.marionette.absolute_url("test.html")
self.marionette.navigate(test_html)
self.marionette.timeouts("script", 10000)
self.assertRaises(ScriptTimeoutException, self.marionette.execute_async_script, "var x = 1;")
def test_no_timeout_settimeout(self):
test_html = self.marionette.absolute_url("test.html")
self.marionette.navigate(test_html)
self.marionette.timeouts("script", 10000)
self.assertTrue(self.marionette.execute_async_script("""
var callback = arguments[arguments.length - 1];
setTimeout(function() { callback(true); }, 500);
"""))
| wilebeast/FireFox-OS | B2G/gecko/testing/marionette/client/marionette/tests/unit/test_timeouts.py | Python | apache-2.0 | 2,769 |
# Copyright (c) 2016 Uber Technologies, Inc.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
from __future__ import print_function
from six.moves import range
import logging
import time
import collections
import mock
import pytest
import tornado.gen
import jaeger_client.reporter
from tornado.concurrent import Future
from jaeger_client import Span, SpanContext
from jaeger_client.metrics import LegacyMetricsFactory, Metrics
from jaeger_client.utils import ErrorReporter
from tornado.ioloop import IOLoop
from tornado.testing import AsyncTestCase, gen_test
from jaeger_client.reporter import Reporter
from jaeger_client.ioloop_util import future_result
def test_null_reporter():
reporter = jaeger_client.reporter.NullReporter()
reporter.report_span({})
f = reporter.close()
f.result()
def test_in_memory_reporter():
reporter = jaeger_client.reporter.InMemoryReporter()
reporter.report_span({})
f = reporter.close()
f.result()
spans = reporter.get_spans()
assert [{}] == spans
def test_logging_reporter():
log_mock = mock.MagicMock()
reporter = jaeger_client.reporter.LoggingReporter(logger=log_mock)
reporter.report_span({})
log_mock.info.assert_called_with('Reporting span %s', {})
reporter.close().result()
def test_composite_reporter():
reporter = jaeger_client.reporter.CompositeReporter(
jaeger_client.reporter.NullReporter(),
jaeger_client.reporter.LoggingReporter())
with mock.patch('jaeger_client.reporter.NullReporter.set_process') \
as null_mock:
with mock.patch('jaeger_client.reporter.LoggingReporter.set_process') \
as log_mock:
reporter.set_process('x', {}, 123)
null_mock.assert_called_with('x', {}, 123)
log_mock.assert_called_with('x', {}, 123)
with mock.patch('jaeger_client.reporter.NullReporter.report_span') \
as null_mock:
with mock.patch('jaeger_client.reporter.LoggingReporter.report_span') \
as log_mock:
reporter.report_span({})
null_mock.assert_called_with({})
log_mock.assert_called_with({})
with mock.patch('jaeger_client.reporter.NullReporter.close') \
as null_mock:
with mock.patch('jaeger_client.reporter.LoggingReporter.close') \
as log_mock:
f1 = Future()
f2 = Future()
null_mock.return_value = f1
log_mock.return_value = f2
f = reporter.close()
null_mock.assert_called_once()
log_mock.assert_called_once()
assert not f.done()
f1.set_result(True)
f2.set_result(True)
assert f.done()
class FakeSender(object):
"""
Mock the _send() method of the reporter by capturing requests
and returning incomplete futures that can be completed from
inside the test.
"""
def __init__(self):
self.requests = []
self.futures = []
def __call__(self, spans):
# print('ManualSender called', request)
self.requests.append(spans)
fut = Future()
self.futures.append(fut)
return fut
class HardErrorReporter(object):
def error(self, name, count, *args):
raise ValueError(*args)
FakeTrace = collections.namedtuple(
'FakeTracer', ['ip_address', 'service_name'])
class FakeMetricsFactory(LegacyMetricsFactory):
def __init__(self):
super(FakeMetricsFactory, self).__init__(
Metrics(count=self._incr_count)
)
self.counters = {}
def _incr_count(self, key, value):
self.counters[key] = value + self.counters.get(key, 0)
class ReporterTest(AsyncTestCase):
@pytest.yield_fixture
def thread_loop(self):
yield
@staticmethod
def _new_span(name):
tracer = FakeTrace(ip_address='127.0.0.1',
service_name='reporter_test')
ctx = SpanContext(trace_id=1,
span_id=1,
parent_id=None,
flags=1)
span = Span(context=ctx,
tracer=tracer,
operation_name=name)
span.start_time = time.time()
span.end_time = span.start_time + 0.001 # 1ms
return span
@staticmethod
def _new_reporter(batch_size, flush=None, queue_cap=100):
reporter = Reporter(channel=mock.MagicMock(),
io_loop=IOLoop.current(),
batch_size=batch_size,
flush_interval=flush,
metrics_factory=FakeMetricsFactory(),
error_reporter=HardErrorReporter(),
queue_capacity=queue_cap)
reporter.set_process('service', {}, max_length=0)
sender = FakeSender()
reporter._send = sender
return reporter, sender
@tornado.gen.coroutine
def _wait_for(self, fn):
"""Wait until fn() returns truth, but not longer than 1 second."""
start = time.time()
for i in range(1000):
if fn():
return
yield tornado.gen.sleep(0.001)
print('waited for condition %f seconds' % (time.time() - start))
@gen_test
def test_submit_batch_size_1(self):
reporter, sender = self._new_reporter(batch_size=1)
reporter.report_span(self._new_span('1'))
yield self._wait_for(lambda: len(sender.futures) > 0)
assert 1 == len(sender.futures)
sender.futures[0].set_result(1)
yield reporter.close()
assert 1 == len(sender.futures)
# send after close
span_dropped_key = 'jaeger:reporter_spans.result_dropped'
assert span_dropped_key not in reporter.metrics_factory.counters
reporter.report_span(self._new_span('1'))
assert 1 == reporter.metrics_factory.counters[span_dropped_key]
@gen_test
def test_submit_failure(self):
reporter, sender = self._new_reporter(batch_size=1)
reporter.error_reporter = ErrorReporter(
metrics=Metrics(), logger=logging.getLogger())
reporter_failure_key = 'jaeger:reporter_spans.result_err'
assert reporter_failure_key not in reporter.metrics_factory.counters
# simulate exception in send
reporter._send = mock.MagicMock(side_effect=ValueError())
reporter.report_span(self._new_span('1'))
yield self._wait_for(
lambda: reporter_failure_key in reporter.metrics_factory.counters)
assert 1 == reporter.metrics_factory.counters.get(reporter_failure_key)
# silly test, for code coverage only
yield reporter._submit([])
@gen_test
def test_submit_queue_full_batch_size_1(self):
reporter, sender = self._new_reporter(batch_size=1, queue_cap=1)
reporter.report_span(self._new_span('1'))
yield self._wait_for(lambda: len(sender.futures) > 0)
assert 1 == len(sender.futures)
# the consumer is blocked on a future, so won't drain the queue
reporter.report_span(self._new_span('2'))
span_dropped_key = 'jaeger:reporter_spans.result_dropped'
assert span_dropped_key not in reporter.metrics_factory.counters
reporter.report_span(self._new_span('3'))
yield self._wait_for(
lambda: span_dropped_key in reporter.metrics_factory.counters
)
assert 1 == reporter.metrics_factory.counters.get(span_dropped_key)
# let it drain the queue
sender.futures[0].set_result(1)
yield self._wait_for(lambda: len(sender.futures) > 1)
assert 2 == len(sender.futures)
sender.futures[1].set_result(1)
yield reporter.close()
@gen_test
def test_submit_batch_size_2(self):
reporter, sender = self._new_reporter(batch_size=2, flush=0.01)
reporter.report_span(self._new_span('1'))
yield tornado.gen.sleep(0.001)
assert 0 == len(sender.futures)
reporter.report_span(self._new_span('2'))
yield self._wait_for(lambda: len(sender.futures) > 0)
assert 1 == len(sender.futures)
assert 2 == len(sender.requests[0].spans)
sender.futures[0].set_result(1)
# 3rd span will not be submitted right away, but after `flush` interval
reporter.report_span(self._new_span('3'))
yield tornado.gen.sleep(0.001)
assert 1 == len(sender.futures)
yield tornado.gen.sleep(0.001)
assert 1 == len(sender.futures)
yield tornado.gen.sleep(0.01)
assert 2 == len(sender.futures)
sender.futures[1].set_result(1)
yield reporter.close()
@gen_test
def test_close_drains_queue(self):
reporter, sender = self._new_reporter(batch_size=1, flush=0.050)
reporter.report_span(self._new_span('0'))
yield self._wait_for(lambda: len(sender.futures) > 0)
assert 1 == len(sender.futures)
# now that the consumer is blocked on the first future.
# let's reset Send to actually respond right away
# and flood the queue with messages
count = [0]
def send(_):
count[0] += 1
return future_result(True)
reporter._send = send
reporter.batch_size = 3
for i in range(10):
reporter.report_span(self._new_span('%s' % i))
assert reporter.queue.qsize() == 10, 'queued 10 spans'
# now unblock consumer
sender.futures[0].set_result(1)
yield self._wait_for(lambda: count[0] > 2)
assert count[0] == 3, '9 out of 10 spans submitted in 3 batches'
assert reporter.queue._unfinished_tasks == 1, 'one span still pending'
yield reporter.close()
assert reporter.queue.qsize() == 0, 'all spans drained'
assert count[0] == 4, 'last span submitted in one extrac batch'
| kawamon/hue | desktop/core/ext-py/jaeger-client-4.0.0/tests/test_reporter.py | Python | apache-2.0 | 10,447 |
# Copyright 2017 Battelle Energy Alliance, LLC
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
import math
import numpy
def run(self, Input):
number_of_steps = 16
self.time = numpy.zeros(number_of_steps)
#Get inputs
Gauss1 = Input["Gauss1"]
auxBackupTimeDist = Input["auxBackupTimeDist"]
Gauss2 = Input["Gauss2"]
CladFailureDist = Input["CladFailureDist"]
self.out1 = numpy.zeros(number_of_steps)
self.out2 = numpy.zeros(number_of_steps)
for i in range(len(self.time)):
self.time[i] = 0.25*float(i)
time = self.time[i]
#calculate outputs
self.out1[i] = time + Gauss1+auxBackupTimeDist + Gauss2 + CladFailureDist
self.out2[i] = time*Gauss1*auxBackupTimeDist*Gauss2*CladFailureDist
| idaholab/raven | tests/framework/Databases/HDF5/test_2steps/two_steps.py | Python | apache-2.0 | 1,215 |
"""The lookin integration sensor platform."""
from __future__ import annotations
import logging
from homeassistant.components.sensor import (
DEVICE_CLASS_HUMIDITY,
DEVICE_CLASS_TEMPERATURE,
STATE_CLASS_MEASUREMENT,
SensorEntity,
SensorEntityDescription,
)
from homeassistant.config_entries import ConfigEntry
from homeassistant.const import PERCENTAGE, TEMP_CELSIUS
from homeassistant.core import HomeAssistant
from homeassistant.helpers.entity_platform import AddEntitiesCallback
from .const import DOMAIN
from .entity import LookinDeviceCoordinatorEntity
from .models import LookinData
LOGGER = logging.getLogger(__name__)
SENSOR_TYPES: tuple[SensorEntityDescription, ...] = (
SensorEntityDescription(
key="temperature",
name="Temperature",
native_unit_of_measurement=TEMP_CELSIUS,
device_class=DEVICE_CLASS_TEMPERATURE,
state_class=STATE_CLASS_MEASUREMENT,
),
SensorEntityDescription(
key="humidity",
name="Humidity",
native_unit_of_measurement=PERCENTAGE,
device_class=DEVICE_CLASS_HUMIDITY,
state_class=STATE_CLASS_MEASUREMENT,
),
)
async def async_setup_entry(
hass: HomeAssistant,
config_entry: ConfigEntry,
async_add_entities: AddEntitiesCallback,
) -> None:
"""Set up lookin sensors from the config entry."""
lookin_data: LookinData = hass.data[DOMAIN][config_entry.entry_id]
async_add_entities(
[LookinSensorEntity(description, lookin_data) for description in SENSOR_TYPES]
)
class LookinSensorEntity(LookinDeviceCoordinatorEntity, SensorEntity):
"""A lookin device sensor entity."""
def __init__(
self, description: SensorEntityDescription, lookin_data: LookinData
) -> None:
"""Init the lookin sensor entity."""
super().__init__(lookin_data)
self.entity_description = description
self._attr_name = f"{self._lookin_device.name} {description.name}"
self._attr_native_value = getattr(self.coordinator.data, description.key)
self._attr_unique_id = f"{self._lookin_device.id}-{description.key}"
def _handle_coordinator_update(self) -> None:
"""Update the state of the entity."""
self._attr_native_value = getattr(
self.coordinator.data, self.entity_description.key
)
super()._handle_coordinator_update()
| jawilson/home-assistant | homeassistant/components/lookin/sensor.py | Python | apache-2.0 | 2,393 |
"""Xbox Media Player Support."""
from __future__ import annotations
import re
from typing import List
from xbox.webapi.api.client import XboxLiveClient
from xbox.webapi.api.provider.catalog.models import Image
from xbox.webapi.api.provider.smartglass.models import (
PlaybackState,
PowerState,
SmartglassConsole,
SmartglassConsoleList,
VolumeDirection,
)
from homeassistant.components.media_player import MediaPlayerEntity
from homeassistant.components.media_player.const import (
MEDIA_TYPE_APP,
MEDIA_TYPE_GAME,
SUPPORT_BROWSE_MEDIA,
SUPPORT_NEXT_TRACK,
SUPPORT_PAUSE,
SUPPORT_PLAY,
SUPPORT_PLAY_MEDIA,
SUPPORT_PREVIOUS_TRACK,
SUPPORT_TURN_OFF,
SUPPORT_TURN_ON,
SUPPORT_VOLUME_MUTE,
SUPPORT_VOLUME_STEP,
)
from homeassistant.const import STATE_OFF, STATE_ON, STATE_PAUSED, STATE_PLAYING
from homeassistant.helpers.update_coordinator import CoordinatorEntity
from . import ConsoleData, XboxUpdateCoordinator
from .browse_media import build_item_response
from .const import DOMAIN
SUPPORT_XBOX = (
SUPPORT_TURN_ON
| SUPPORT_TURN_OFF
| SUPPORT_PREVIOUS_TRACK
| SUPPORT_NEXT_TRACK
| SUPPORT_PLAY
| SUPPORT_PAUSE
| SUPPORT_VOLUME_STEP
| SUPPORT_VOLUME_MUTE
| SUPPORT_BROWSE_MEDIA
| SUPPORT_PLAY_MEDIA
)
XBOX_STATE_MAP = {
PlaybackState.Playing: STATE_PLAYING,
PlaybackState.Paused: STATE_PAUSED,
PowerState.On: STATE_ON,
PowerState.SystemUpdate: STATE_OFF,
PowerState.ConnectedStandby: STATE_OFF,
PowerState.Off: STATE_OFF,
PowerState.Unknown: None,
}
async def async_setup_entry(hass, entry, async_add_entities):
"""Set up Xbox media_player from a config entry."""
client: XboxLiveClient = hass.data[DOMAIN][entry.entry_id]["client"]
consoles: SmartglassConsoleList = hass.data[DOMAIN][entry.entry_id]["consoles"]
coordinator: XboxUpdateCoordinator = hass.data[DOMAIN][entry.entry_id][
"coordinator"
]
async_add_entities(
[XboxMediaPlayer(client, console, coordinator) for console in consoles.result]
)
class XboxMediaPlayer(CoordinatorEntity, MediaPlayerEntity):
"""Representation of an Xbox Media Player."""
def __init__(
self,
client: XboxLiveClient,
console: SmartglassConsole,
coordinator: XboxUpdateCoordinator,
) -> None:
"""Initialize the Xbox Media Player."""
super().__init__(coordinator)
self.client: XboxLiveClient = client
self._console: SmartglassConsole = console
@property
def name(self):
"""Return the device name."""
return self._console.name
@property
def unique_id(self):
"""Console device ID."""
return self._console.id
@property
def data(self) -> ConsoleData:
"""Return coordinator data for this console."""
return self.coordinator.data.consoles[self._console.id]
@property
def state(self):
"""State of the player."""
status = self.data.status
if status.playback_state in XBOX_STATE_MAP:
return XBOX_STATE_MAP[status.playback_state]
return XBOX_STATE_MAP[status.power_state]
@property
def supported_features(self):
"""Flag media player features that are supported."""
if self.state not in [STATE_PLAYING, STATE_PAUSED]:
return SUPPORT_XBOX & ~SUPPORT_NEXT_TRACK & ~SUPPORT_PREVIOUS_TRACK
return SUPPORT_XBOX
@property
def media_content_type(self):
"""Media content type."""
app_details = self.data.app_details
if app_details and app_details.product_family == "Games":
return MEDIA_TYPE_GAME
return MEDIA_TYPE_APP
@property
def media_title(self):
"""Title of current playing media."""
app_details = self.data.app_details
if not app_details:
return None
return (
app_details.localized_properties[0].product_title
or app_details.localized_properties[0].short_title
)
@property
def media_image_url(self):
"""Image url of current playing media."""
app_details = self.data.app_details
if not app_details:
return None
image = _find_media_image(app_details.localized_properties[0].images)
if not image:
return None
url = image.uri
if url[0] == "/":
url = f"http:{url}"
return url
@property
def media_image_remotely_accessible(self) -> bool:
"""If the image url is remotely accessible."""
return True
async def async_turn_on(self):
"""Turn the media player on."""
await self.client.smartglass.wake_up(self._console.id)
async def async_turn_off(self):
"""Turn the media player off."""
await self.client.smartglass.turn_off(self._console.id)
async def async_mute_volume(self, mute):
"""Mute the volume."""
if mute:
await self.client.smartglass.mute(self._console.id)
else:
await self.client.smartglass.unmute(self._console.id)
async def async_volume_up(self):
"""Turn volume up for media player."""
await self.client.smartglass.volume(self._console.id, VolumeDirection.Up)
async def async_volume_down(self):
"""Turn volume down for media player."""
await self.client.smartglass.volume(self._console.id, VolumeDirection.Down)
async def async_media_play(self):
"""Send play command."""
await self.client.smartglass.play(self._console.id)
async def async_media_pause(self):
"""Send pause command."""
await self.client.smartglass.pause(self._console.id)
async def async_media_previous_track(self):
"""Send previous track command."""
await self.client.smartglass.previous(self._console.id)
async def async_media_next_track(self):
"""Send next track command."""
await self.client.smartglass.next(self._console.id)
async def async_browse_media(self, media_content_type=None, media_content_id=None):
"""Implement the websocket media browsing helper."""
return await build_item_response(
self.client,
self._console.id,
self.data.status.is_tv_configured,
media_content_type,
media_content_id,
)
async def async_play_media(self, media_type, media_id, **kwargs):
"""Launch an app on the Xbox."""
if media_id == "Home":
await self.client.smartglass.go_home(self._console.id)
elif media_id == "TV":
await self.client.smartglass.show_tv_guide(self._console.id)
else:
await self.client.smartglass.launch_app(self._console.id, media_id)
@property
def device_info(self):
"""Return a device description for device registry."""
# Turns "XboxOneX" into "Xbox One X" for display
matches = re.finditer(
".+?(?:(?<=[a-z])(?=[A-Z])|(?<=[A-Z])(?=[A-Z][a-z])|$)",
self._console.console_type,
)
model = " ".join([m.group(0) for m in matches])
return {
"identifiers": {(DOMAIN, self._console.id)},
"name": self._console.name,
"manufacturer": "Microsoft",
"model": model,
}
def _find_media_image(images=List[Image]) -> Image | None:
purpose_order = ["FeaturePromotionalSquareArt", "Tile", "Logo", "BoxArt"]
for purpose in purpose_order:
for image in images:
if (
image.image_purpose == purpose
and image.width == image.height
and image.width >= 300
):
return image
return None
| w1ll1am23/home-assistant | homeassistant/components/xbox/media_player.py | Python | apache-2.0 | 7,802 |
import unittest
import wx
from editor.fakeplugin import FakePlugin
from robot.utils.asserts import assert_true
from robotide.controller.macrocontrollers import TestCaseController
from robotide.editor.macroeditors import TestCaseEditor
TestCaseEditor._populate = lambda self: None
class IncredibleMock(object):
def __getattr__(self, item):
return self
def __call__(self, *args, **kwargs):
return self
class MockKwEditor(object):
_expect = None
_called = None
def __getattr__(self, item):
self._active_item = item
return self
def __call__(self, *args, **kwargs):
self._called = self._active_item
def is_to_be_called(self):
self._expect = self._active_item
def has_been_called(self):
return self._active_item == self._expect == self._called
class MacroEditorTest(unittest.TestCase):
def setUp(self):
controller = TestCaseController(IncredibleMock(), IncredibleMock())
plugin = FakePlugin({}, controller)
self.tc_editor = TestCaseEditor(
plugin, wx.Frame(None), controller, None)
def test_delegation_to_kw_editor(self):
for method, kw_method in \
[('save', 'save'),
('undo', 'OnUndo'),
('redo', 'OnRedo'),
('cut', 'OnCut'),
('copy', 'OnCopy'),
('paste', 'OnPaste'),
('insert', 'OnInsert'),
('insert_rows', 'OnInsertRows'),
('delete_rows', 'OnDeleteRows'),
('delete', 'OnDelete'),
('comment', 'OnCommentRows'),
('uncomment', 'OnUncommentRows'),
('show_content_assist', 'show_content_assist')]:
kw_mock = MockKwEditor()
self.tc_editor.kweditor = kw_mock
getattr(kw_mock, kw_method).is_to_be_called()
getattr(self.tc_editor, method)()
assert_true(getattr(kw_mock, kw_method).has_been_called(),
'Should have called "%s" when calling "%s"' %
(kw_method, method))
| caio2k/RIDE | utest/editor/test_macroeditors.py | Python | apache-2.0 | 2,087 |
from plenum.test.view_change_service.helper import check_view_change_adding_new_node
def test_view_change_while_adding_new_node_2_slow_commit(looper, tdir, tconf, allPluginsPath,
txnPoolNodeSet,
sdk_pool_handle,
sdk_wallet_client,
sdk_wallet_steward):
check_view_change_adding_new_node(looper, tdir, tconf, allPluginsPath,
txnPoolNodeSet,
sdk_pool_handle,
sdk_wallet_client,
sdk_wallet_steward,
slow_nodes=[txnPoolNodeSet[1], txnPoolNodeSet[2]],
delay_pre_prepare=False,
delay_commit=True,
trigger_view_change_manually=True)
| evernym/zeno | plenum/test/view_change_service/test_view_change_while_adding_new_node_2_slow_commit.py | Python | apache-2.0 | 1,063 |
# Copyright 2012 OpenStack Foundation
# All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License"); you may
# not use this file except in compliance with the License. You may obtain
# a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
# License for the specific language governing permissions and limitations
# under the License.
from tempest.api.compute.security_groups import base
from tempest import config
from tempest import test
CONF = config.CONF
class SecurityGroupRulesTestJSON(base.BaseSecurityGroupsTest):
@classmethod
def resource_setup(cls):
super(SecurityGroupRulesTestJSON, cls).resource_setup()
cls.client = cls.security_groups_client
cls.neutron_available = CONF.service_available.neutron
cls.ip_protocol = 'tcp'
cls.from_port = 22
cls.to_port = 22
def setUp(cls):
super(SecurityGroupRulesTestJSON, cls).setUp()
from_port = cls.from_port
to_port = cls.to_port
group = {}
ip_range = {}
cls.expected = {
'id': None,
'parent_group_id': None,
'ip_protocol': cls.ip_protocol,
'from_port': from_port,
'to_port': to_port,
'ip_range': ip_range,
'group': group
}
def _check_expected_response(self, actual_rule):
for key in self.expected:
if key == 'id':
continue
self.assertEqual(self.expected[key], actual_rule[key],
"Miss-matched key is %s" % key)
@test.attr(type='smoke')
@test.services('network')
def test_security_group_rules_create(self):
# Positive test: Creation of Security Group rule
# should be successful
# Creating a Security Group to add rules to it
security_group = self.create_security_group()
securitygroup_id = security_group['id']
# Adding rules to the created Security Group
rule = \
self.client.create_security_group_rule(securitygroup_id,
self.ip_protocol,
self.from_port,
self.to_port)
self.expected['parent_group_id'] = securitygroup_id
self.expected['ip_range'] = {'cidr': '0.0.0.0/0'}
self._check_expected_response(rule)
@test.attr(type='smoke')
@test.services('network')
def test_security_group_rules_create_with_optional_cidr(self):
# Positive test: Creation of Security Group rule
# with optional argument cidr
# should be successful
# Creating a Security Group to add rules to it
security_group = self.create_security_group()
parent_group_id = security_group['id']
# Adding rules to the created Security Group with optional cidr
cidr = '10.2.3.124/24'
rule = \
self.client.create_security_group_rule(parent_group_id,
self.ip_protocol,
self.from_port,
self.to_port,
cidr=cidr)
self.expected['parent_group_id'] = parent_group_id
self.expected['ip_range'] = {'cidr': cidr}
self._check_expected_response(rule)
@test.attr(type='smoke')
@test.services('network')
def test_security_group_rules_create_with_optional_group_id(self):
# Positive test: Creation of Security Group rule
# with optional argument group_id
# should be successful
# Creating a Security Group to add rules to it
security_group = self.create_security_group()
parent_group_id = security_group['id']
# Creating a Security Group so as to assign group_id to the rule
security_group = self.create_security_group()
group_id = security_group['id']
group_name = security_group['name']
# Adding rules to the created Security Group with optional group_id
rule = \
self.client.create_security_group_rule(parent_group_id,
self.ip_protocol,
self.from_port,
self.to_port,
group_id=group_id)
self.expected['parent_group_id'] = parent_group_id
self.expected['group'] = {'tenant_id': self.client.tenant_id,
'name': group_name}
self._check_expected_response(rule)
@test.attr(type='smoke')
@test.services('network')
def test_security_group_rules_list(self):
# Positive test: Created Security Group rules should be
# in the list of all rules
# Creating a Security Group to add rules to it
security_group = self.create_security_group()
securitygroup_id = security_group['id']
# Add a first rule to the created Security Group
rule = \
self.client.create_security_group_rule(securitygroup_id,
self.ip_protocol,
self.from_port,
self.to_port)
rule1_id = rule['id']
# Add a second rule to the created Security Group
ip_protocol2 = 'icmp'
from_port2 = -1
to_port2 = -1
rule = \
self.client.create_security_group_rule(securitygroup_id,
ip_protocol2,
from_port2, to_port2)
rule2_id = rule['id']
# Delete the Security Group rule2 at the end of this method
self.addCleanup(self.client.delete_security_group_rule, rule2_id)
# Get rules of the created Security Group
rules = \
self.client.list_security_group_rules(securitygroup_id)
self.assertTrue(any([i for i in rules if i['id'] == rule1_id]))
self.assertTrue(any([i for i in rules if i['id'] == rule2_id]))
@test.attr(type='smoke')
@test.services('network')
def test_security_group_rules_delete_when_peer_group_deleted(self):
# Positive test:rule will delete when peer group deleting
# Creating a Security Group to add rules to it
security_group = self.create_security_group()
sg1_id = security_group['id']
# Creating other Security Group to access to group1
security_group = self.create_security_group()
sg2_id = security_group['id']
# Adding rules to the Group1
self.client.create_security_group_rule(sg1_id,
self.ip_protocol,
self.from_port,
self.to_port,
group_id=sg2_id)
# Delete group2
self.client.delete_security_group(sg2_id)
# Get rules of the Group1
rules = \
self.client.list_security_group_rules(sg1_id)
# The group1 has no rules because group2 has deleted
self.assertEqual(0, len(rules))
| jamielennox/tempest | tempest/api/compute/security_groups/test_security_group_rules.py | Python | apache-2.0 | 7,690 |
from .base import *
import os
DEBUG = True
ALLOWED_HOSTS = ['*']
INTERNAL_IPS = ['127.0.0.1']
# Set the path to the location of the content files for python.org
# For example,
# PYTHON_ORG_CONTENT_SVN_PATH = '/Users/flavio/working_copies/beta.python.org/build/data'
PYTHON_ORG_CONTENT_SVN_PATH = ''
DATABASES = {
'default': config(
'DATABASE_URL',
default='postgres:///pythondotorg',
cast=dj_database_url_parser
)
}
HAYSTACK_SEARCHBOX_SSL_URL = config(
'SEARCHBOX_SSL_URL',
default='http://127.0.0.1:9200/'
)
HAYSTACK_CONNECTIONS = {
'default': {
'ENGINE': 'haystack.backends.elasticsearch5_backend.Elasticsearch5SearchEngine',
'URL': HAYSTACK_SEARCHBOX_SSL_URL,
'INDEX_NAME': 'haystack',
},
}
EMAIL_BACKEND = 'django.core.mail.backends.console.EmailBackend'
# Set the local pep repository path to fetch PEPs from,
# or none to fallback to the tarball specified by PEP_ARTIFACT_URL.
PEP_REPO_PATH = config('PEP_REPO_PATH', default=None) # directory path or None
# Set the path to where to fetch PEP artifacts from.
# The value can be a local path or a remote URL.
# Ignored if PEP_REPO_PATH is set.
PEP_ARTIFACT_URL = os.path.join(BASE, 'peps/tests/peps.tar.gz')
# Use Dummy SASS compiler to avoid performance issues and remove the need to
# have a sass compiler installed at all during local development if you aren't
# adjusting the CSS at all. Comment this out or adjust it to suit your local
# environment needs if you are working with the CSS.
# PIPELINE['COMPILERS'] = (
# 'pydotorg.compilers.DummySASSCompiler',
# )
# Pass '-XssNNNNNk' to 'java' if you get 'java.lang.StackOverflowError' with
# yui-compressor.
# PIPELINE['YUI_BINARY'] = '/usr/bin/java -Xss200048k -jar /usr/share/yui-compressor/yui-compressor.jar'
INSTALLED_APPS += [
'debug_toolbar',
]
MIDDLEWARE += [
'debug_toolbar.middleware.DebugToolbarMiddleware',
]
CACHES = {
'default': {
'BACKEND': 'django.core.cache.backends.dummy.DummyCache',
}
}
REST_FRAMEWORK['DEFAULT_RENDERER_CLASSES'] += (
'rest_framework.renderers.BrowsableAPIRenderer',
)
| manhhomienbienthuy/pythondotorg | pydotorg/settings/local.py | Python | apache-2.0 | 2,135 |
from dtest import Tester
from tools import putget
class TestMultiDCPutGet(Tester):
def putget_2dc_rf1_test(self):
""" Simple put-get test for 2 DC with one node each (RF=1) [catches #3539] """
cluster = self.cluster
cluster.populate([1, 1]).start()
session = self.patient_cql_connection(cluster.nodelist()[0])
self.create_ks(session, 'ks', {'dc1': 1, 'dc2': 1})
self.create_cf(session, 'cf')
putget(cluster, session)
def putget_2dc_rf2_test(self):
""" Simple put-get test for 2 DC with 2 node each (RF=2) -- tests cross-DC efficient writes """
cluster = self.cluster
cluster.populate([2, 2]).start()
session = self.patient_cql_connection(cluster.nodelist()[0])
self.create_ks(session, 'ks', {'dc1': 2, 'dc2': 2})
self.create_cf(session, 'cf')
putget(cluster, session)
| mambocab/cassandra-dtest | multidc_putget_test.py | Python | apache-2.0 | 896 |
# Copyright 2017 The TensorFlow Authors. All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# ==============================================================================
"""Collection of parsers which are shared among the official models.
The parsers in this module are intended to be used as parents to all arg
parsers in official models. For instance, one might define a new class:
class ExampleParser(argparse.ArgumentParser):
def __init__(self):
super(ExampleParser, self).__init__(parents=[
arg_parsers.LocationParser(data_dir=True, model_dir=True),
arg_parsers.DummyParser(use_synthetic_data=True),
])
self.add_argument(
"--application_specific_arg", "-asa", type=int, default=123,
help="[default: %(default)s] This arg is application specific.",
metavar="<ASA>"
)
Notes about add_argument():
Argparse will automatically template in default values in help messages if
the "%(default)s" string appears in the message. Using the example above:
parser = ExampleParser()
parser.set_defaults(application_specific_arg=3141592)
parser.parse_args(["-h"])
When the help text is generated, it will display 3141592 to the user. (Even
though the default was 123 when the flag was created.)
The metavar variable determines how the flag will appear in help text. If
not specified, the convention is to use name.upper(). Thus rather than:
--app_specific_arg APP_SPECIFIC_ARG, -asa APP_SPECIFIC_ARG
if metavar="<ASA>" is set, the user sees:
--app_specific_arg <ASA>, -asa <ASA>
"""
from __future__ import absolute_import
from __future__ import division
from __future__ import print_function
import argparse
import tensorflow as tf
# Map string to (TensorFlow dtype, default loss scale)
DTYPE_MAP = {
"fp16": (tf.float16, 128),
"fp32": (tf.float32, 1),
}
def parse_dtype_info(flags):
"""Convert dtype string to tf dtype, and set loss_scale default as needed.
Args:
flags: namespace object returned by arg parser.
Raises:
ValueError: If an invalid dtype is provided.
"""
if flags.dtype in (i[0] for i in DTYPE_MAP.values()):
return # Make function idempotent
try:
flags.dtype, default_loss_scale = DTYPE_MAP[flags.dtype]
except KeyError:
raise ValueError("Invalid dtype: {}".format(flags.dtype))
flags.loss_scale = flags.loss_scale or default_loss_scale
class BaseParser(argparse.ArgumentParser):
"""Parser to contain flags which will be nearly universal across models.
Args:
add_help: Create the "--help" flag. False if class instance is a parent.
data_dir: Create a flag for specifying the input data directory.
model_dir: Create a flag for specifying the model file directory.
train_epochs: Create a flag to specify the number of training epochs.
epochs_between_evals: Create a flag to specify the frequency of testing.
stop_threshold: Create a flag to specify a threshold accuracy or other
eval metric which should trigger the end of training.
batch_size: Create a flag to specify the global batch size.
multi_gpu: Create a flag to allow the use of all available GPUs.
num_gpu: Create a flag to specify the number of GPUs used.
hooks: Create a flag to specify hooks for logging.
"""
def __init__(self, add_help=False, data_dir=True, model_dir=True,
train_epochs=True, epochs_between_evals=True,
stop_threshold=True, batch_size=True,
multi_gpu=False, num_gpu=True, hooks=True):
super(BaseParser, self).__init__(add_help=add_help)
if data_dir:
self.add_argument(
"--data_dir", "-dd", default="/tmp",
help="[default: %(default)s] The location of the input data.",
metavar="<DD>",
)
if model_dir:
self.add_argument(
"--model_dir", "-md", default="/tmp",
help="[default: %(default)s] The location of the model checkpoint "
"files.",
metavar="<MD>",
)
if train_epochs:
self.add_argument(
"--train_epochs", "-te", type=int, default=1,
help="[default: %(default)s] The number of epochs used to train.",
metavar="<TE>"
)
if epochs_between_evals:
self.add_argument(
"--epochs_between_evals", "-ebe", type=int, default=1,
help="[default: %(default)s] The number of training epochs to run "
"between evaluations.",
metavar="<EBE>"
)
if stop_threshold:
self.add_argument(
"--stop_threshold", "-st", type=float, default=None,
help="[default: %(default)s] If passed, training will stop at "
"the earlier of train_epochs and when the evaluation metric is "
"greater than or equal to stop_threshold.",
metavar="<ST>"
)
if batch_size:
self.add_argument(
"--batch_size", "-bs", type=int, default=32,
help="[default: %(default)s] Global batch size for training and "
"evaluation.",
metavar="<BS>"
)
assert not (multi_gpu and num_gpu)
if multi_gpu:
self.add_argument(
"--multi_gpu", action="store_true",
help="If set, run across all available GPUs."
)
if num_gpu:
self.add_argument(
"--num_gpus", "-ng",
type=int,
default=1 if tf.test.is_built_with_cuda() else 0,
help="[default: %(default)s] How many GPUs to use with the "
"DistributionStrategies API. The default is 1 if TensorFlow was"
"built with CUDA, and 0 otherwise.",
metavar="<NG>"
)
if hooks:
self.add_argument(
"--hooks", "-hk", nargs="+", default=["LoggingTensorHook"],
help="[default: %(default)s] A list of strings to specify the names "
"of train hooks. "
"Example: --hooks LoggingTensorHook ExamplesPerSecondHook. "
"Allowed hook names (case-insensitive): LoggingTensorHook, "
"ProfilerHook, ExamplesPerSecondHook, LoggingMetricHook."
"See official.utils.logs.hooks_helper for details.",
metavar="<HK>"
)
class PerformanceParser(argparse.ArgumentParser):
"""Default parser for specifying performance tuning arguments.
Args:
add_help: Create the "--help" flag. False if class instance is a parent.
num_parallel_calls: Create a flag to specify parallelism of data loading.
inter_op: Create a flag to allow specification of inter op threads.
intra_op: Create a flag to allow specification of intra op threads.
"""
def __init__(self, add_help=False, num_parallel_calls=True, inter_op=True,
intra_op=True, use_synthetic_data=True, max_train_steps=True,
dtype=True):
super(PerformanceParser, self).__init__(add_help=add_help)
if num_parallel_calls:
self.add_argument(
"--num_parallel_calls", "-npc",
type=int, default=5,
help="[default: %(default)s] The number of records that are "
"processed in parallel during input processing. This can be "
"optimized per data set but for generally homogeneous data "
"sets, should be approximately the number of available CPU "
"cores.",
metavar="<NPC>"
)
if inter_op:
self.add_argument(
"--inter_op_parallelism_threads", "-inter",
type=int, default=0,
help="[default: %(default)s Number of inter_op_parallelism_threads "
"to use for CPU. See TensorFlow config.proto for details.",
metavar="<INTER>"
)
if intra_op:
self.add_argument(
"--intra_op_parallelism_threads", "-intra",
type=int, default=0,
help="[default: %(default)s Number of intra_op_parallelism_threads "
"to use for CPU. See TensorFlow config.proto for details.",
metavar="<INTRA>"
)
if use_synthetic_data:
self.add_argument(
"--use_synthetic_data", "-synth",
action="store_true",
help="If set, use fake data (zeroes) instead of a real dataset. "
"This mode is useful for performance debugging, as it removes "
"input processing steps, but will not learn anything."
)
if max_train_steps:
self.add_argument(
"--max_train_steps", "-mts", type=int, default=None,
help="[default: %(default)s] The model will stop training if the "
"global_step reaches this value. If not set, training will run"
"until the specified number of epochs have run as usual. It is"
"generally recommended to set --train_epochs=1 when using this"
"flag.",
metavar="<MTS>"
)
if dtype:
self.add_argument(
"--dtype", "-dt",
default="fp32",
choices=list(DTYPE_MAP.keys()),
help="[default: %(default)s] {%(choices)s} The TensorFlow datatype "
"used for calculations. Variables may be cast to a higher"
"precision on a case-by-case basis for numerical stability.",
metavar="<DT>"
)
self.add_argument(
"--loss_scale", "-ls",
type=int,
help="[default: %(default)s] The amount to scale the loss by when "
"the model is run. Before gradients are computed, the loss is "
"multiplied by the loss scale, making all gradients loss_scale "
"times larger. To adjust for this, gradients are divided by the "
"loss scale before being applied to variables. This is "
"mathematically equivalent to training without a loss scale, "
"but the loss scale helps avoid some intermediate gradients "
"from underflowing to zero. If not provided the default for "
"fp16 is 128 and 1 for all other dtypes.",
)
class ImageModelParser(argparse.ArgumentParser):
"""Default parser for specification image specific behavior.
Args:
add_help: Create the "--help" flag. False if class instance is a parent.
data_format: Create a flag to specify image axis convention.
"""
def __init__(self, add_help=False, data_format=True):
super(ImageModelParser, self).__init__(add_help=add_help)
if data_format:
self.add_argument(
"--data_format", "-df",
default=None,
choices=["channels_first", "channels_last"],
help="A flag to override the data format used in the model. "
"channels_first provides a performance boost on GPU but is not "
"always compatible with CPU. If left unspecified, the data "
"format will be chosen automatically based on whether TensorFlow"
"was built for CPU or GPU.",
metavar="<CF>"
)
class ExportParser(argparse.ArgumentParser):
"""Parsing options for exporting saved models or other graph defs.
This is a separate parser for now, but should be made part of BaseParser
once all models are brought up to speed.
Args:
add_help: Create the "--help" flag. False if class instance is a parent.
export_dir: Create a flag to specify where a SavedModel should be exported.
"""
def __init__(self, add_help=False, export_dir=True):
super(ExportParser, self).__init__(add_help=add_help)
if export_dir:
self.add_argument(
"--export_dir", "-ed",
help="[default: %(default)s] If set, a SavedModel serialization of "
"the model will be exported to this directory at the end of "
"training. See the README for more details and relevant links.",
metavar="<ED>"
)
class BenchmarkParser(argparse.ArgumentParser):
"""Default parser for benchmark logging.
Args:
add_help: Create the "--help" flag. False if class instance is a parent.
benchmark_log_dir: Create a flag to specify location for benchmark logging.
"""
def __init__(self, add_help=False, benchmark_log_dir=True,
bigquery_uploader=True):
super(BenchmarkParser, self).__init__(add_help=add_help)
if benchmark_log_dir:
self.add_argument(
"--benchmark_log_dir", "-bld", default=None,
help="[default: %(default)s] The location of the benchmark logging.",
metavar="<BLD>"
)
if bigquery_uploader:
self.add_argument(
"--gcp_project", "-gp", default=None,
help="[default: %(default)s] The GCP project name where the benchmark"
" will be uploaded.",
metavar="<GP>"
)
self.add_argument(
"--bigquery_data_set", "-bds", default="test_benchmark",
help="[default: %(default)s] The Bigquery dataset name where the"
" benchmark will be uploaded.",
metavar="<BDS>"
)
self.add_argument(
"--bigquery_run_table", "-brt", default="benchmark_run",
help="[default: %(default)s] The Bigquery table name where the"
" benchmark run information will be uploaded.",
metavar="<BRT>"
)
self.add_argument(
"--bigquery_metric_table", "-bmt", default="benchmark_metric",
help="[default: %(default)s] The Bigquery table name where the"
" benchmark metric information will be uploaded.",
metavar="<BMT>"
)
class EagerParser(BaseParser):
"""Remove options not relevant for Eager from the BaseParser."""
def __init__(self, add_help=False, data_dir=True, model_dir=True,
train_epochs=True, batch_size=True):
super(EagerParser, self).__init__(
add_help=add_help, data_dir=data_dir, model_dir=model_dir,
train_epochs=train_epochs, epochs_between_evals=False,
stop_threshold=False, batch_size=batch_size, multi_gpu=False,
hooks=False)
| floydhub/dockerfiles | dl/tensorflow/tests/1.7.0/parsers.py | Python | apache-2.0 | 14,510 |
# Copyright 2012, Red Hat, Inc.
#
# Licensed under the Apache License, Version 2.0 (the "License"); you may
# not use this file except in compliance with the License. You may obtain
# a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
# License for the specific language governing permissions and limitations
# under the License.
"""
Unit Tests for nova.cert.rpcapi
"""
import mock
from oslo_config import cfg
from nova.cert import rpcapi as cert_rpcapi
from nova import context
from nova import test
CONF = cfg.CONF
class CertRpcAPITestCase(test.NoDBTestCase):
def _test_cert_api(self, method, **kwargs):
ctxt = context.RequestContext('fake_user', 'fake_project')
rpcapi = cert_rpcapi.CertAPI()
self.assertIsNotNone(rpcapi.client)
orig_prepare = rpcapi.client.prepare
with test.nested(
mock.patch.object(rpcapi.client, 'call'),
mock.patch.object(rpcapi.client, 'prepare'),
mock.patch.object(rpcapi.client, 'can_send_version'),
) as (
rpc_mock, prepare_mock, csv_mock
):
prepare_mock.return_value = rpcapi.client
rpc_mock.return_value = 'foo'
csv_mock.side_effect = (
lambda v: orig_prepare().can_send_version())
retval = getattr(rpcapi, method)(ctxt, **kwargs)
self.assertEqual(rpc_mock.return_value, retval)
prepare_mock.assert_called_once_with()
rpc_mock.assert_called_once_with(ctxt, method, **kwargs)
def test_revoke_certs_by_user(self):
self._test_cert_api('revoke_certs_by_user', user_id='fake_user_id')
def test_revoke_certs_by_project(self):
self._test_cert_api('revoke_certs_by_project',
project_id='fake_project_id')
def test_revoke_certs_by_user_and_project(self):
self._test_cert_api('revoke_certs_by_user_and_project',
user_id='fake_user_id',
project_id='fake_project_id')
def test_generate_x509_cert(self):
self._test_cert_api('generate_x509_cert',
user_id='fake_user_id',
project_id='fake_project_id')
def test_fetch_ca(self):
self._test_cert_api('fetch_ca', project_id='fake_project_id')
def test_fetch_crl(self):
self._test_cert_api('fetch_crl', project_id='fake_project_id')
def test_decrypt_text(self):
self._test_cert_api('decrypt_text',
project_id='fake_project_id', text='blah')
| hanlind/nova | nova/tests/unit/cert/test_rpcapi.py | Python | apache-2.0 | 2,856 |
def __bootstrap__():
global __bootstrap__, __loader__, __file__
import sys, pkg_resources, imp
__file__ = pkg_resources.resource_filename(__name__, '_psycopg.so')
__loader__ = None; del __bootstrap__, __loader__
imp.load_dynamic(__name__,__file__)
__bootstrap__()
| hexlism/css_platform | sleepyenv/lib/python2.7/site-packages/psycopg2-2.6.1-py2.7-linux-x86_64.egg/psycopg2/_psycopg.py | Python | apache-2.0 | 284 |
# Copyright 2020 Mycroft AI Inc.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
#
from unittest import TestCase
from mycroft.client.speech.mic import NoiseTracker
LOUD_TIME_LIMIT = 2.0 # Must be loud for 2 seconds
SILENCE_TIME_LIMIT = 5.0 # Time out after 5 seconds of silence
SECS_PER_BUFFER = 0.5
MIN_NOISE = 0
MAX_NOISE = 25
class TestNoiseTracker(TestCase):
def test_no_loud_data(self):
"""Check that no loud data generates complete after silence timeout."""
noise_tracker = NoiseTracker(MIN_NOISE, MAX_NOISE, SECS_PER_BUFFER,
LOUD_TIME_LIMIT, SILENCE_TIME_LIMIT)
num_updates_timeout = int(SILENCE_TIME_LIMIT / SECS_PER_BUFFER)
num_low_updates = int(LOUD_TIME_LIMIT / SECS_PER_BUFFER)
for _ in range(num_low_updates):
noise_tracker.update(False)
self.assertFalse(noise_tracker.recording_complete())
remaining_until_low_timeout = num_updates_timeout - num_low_updates
for _ in range(remaining_until_low_timeout):
noise_tracker.update(False)
self.assertFalse(noise_tracker.recording_complete())
noise_tracker.update(False)
self.assertTrue(noise_tracker.recording_complete())
def test_silence_reset(self):
"""Check that no loud data generates complete after silence timeout."""
noise_tracker = NoiseTracker(MIN_NOISE, MAX_NOISE, SECS_PER_BUFFER,
LOUD_TIME_LIMIT, SILENCE_TIME_LIMIT)
num_updates_timeout = int(SILENCE_TIME_LIMIT / SECS_PER_BUFFER)
num_low_updates = int(LOUD_TIME_LIMIT / SECS_PER_BUFFER)
for _ in range(num_low_updates):
noise_tracker.update(False)
# Insert a is_loud=True shall reset the silence tracker
noise_tracker.update(True)
remaining_until_low_timeout = num_updates_timeout - num_low_updates
# Extra is needed for the noise to be reduced down to quiet level
for _ in range(remaining_until_low_timeout + 1):
noise_tracker.update(False)
self.assertFalse(noise_tracker.recording_complete())
# Adding low noise samples to complete the timeout
for _ in range(num_low_updates + 1):
noise_tracker.update(False)
self.assertTrue(noise_tracker.recording_complete())
def test_all_loud_data(self):
"""Check that only loud samples doesn't generate a complete recording.
"""
noise_tracker = NoiseTracker(MIN_NOISE, MAX_NOISE, SECS_PER_BUFFER,
LOUD_TIME_LIMIT, SILENCE_TIME_LIMIT)
num_high_updates = int(LOUD_TIME_LIMIT / SECS_PER_BUFFER) + 1
for _ in range(num_high_updates):
noise_tracker.update(True)
self.assertFalse(noise_tracker.recording_complete())
def test_all_loud_followed_by_silence(self):
"""Check that a long enough high sentence is completed after silence.
"""
noise_tracker = NoiseTracker(MIN_NOISE, MAX_NOISE, SECS_PER_BUFFER,
LOUD_TIME_LIMIT, SILENCE_TIME_LIMIT)
num_high_updates = int(LOUD_TIME_LIMIT / SECS_PER_BUFFER) + 1
for _ in range(num_high_updates):
noise_tracker.update(True)
self.assertFalse(noise_tracker.recording_complete())
while not noise_tracker._quiet_enough():
noise_tracker.update(False)
self.assertTrue(noise_tracker.recording_complete())
| forslund/mycroft-core | test/unittests/client/test_noise_tracker.py | Python | apache-2.0 | 4,000 |
# Copyright 2013 Canonical Corp.
# All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License"); you may
# not use this file except in compliance with the License. You may obtain
# a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
# License for the specific language governing permissions and limitations
# under the License.
import mock
from oslo_config import cfg
from oslo_vmware import exceptions as vexc
from oslo_vmware import vim_util as vutil
from nova import exception
from nova.network import model as network_model
from nova import test
from nova.tests.unit import matchers
from nova.tests.unit import utils
from nova.tests.unit.virt.vmwareapi import fake
from nova.virt.vmwareapi import network_util
from nova.virt.vmwareapi import vif
from nova.virt.vmwareapi import vm_util
CONF = cfg.CONF
class VMwareVifTestCase(test.NoDBTestCase):
def setUp(self):
super(VMwareVifTestCase, self).setUp()
self.flags(vlan_interface='vmnet0', group='vmware')
network = network_model.Network(id=0,
bridge='fa0',
label='fake',
vlan=3,
bridge_interface='eth0',
injected=True)
self.vif = network_model.NetworkInfo([
network_model.VIF(id=None,
address='DE:AD:BE:EF:00:00',
network=network,
type=None,
devname=None,
ovs_interfaceid=None,
rxtx_cap=3)
])[0]
self.session = fake.FakeSession()
self.cluster = None
def tearDown(self):
super(VMwareVifTestCase, self).tearDown()
def test_ensure_vlan_bridge(self):
self.mox.StubOutWithMock(network_util, 'get_network_with_the_name')
self.mox.StubOutWithMock(network_util,
'get_vswitch_for_vlan_interface')
self.mox.StubOutWithMock(network_util,
'check_if_vlan_interface_exists')
self.mox.StubOutWithMock(network_util, 'create_port_group')
network_util.get_network_with_the_name(self.session, 'fa0',
self.cluster).AndReturn(None)
network_util.get_vswitch_for_vlan_interface(self.session, 'vmnet0',
self.cluster).AndReturn('vmnet0')
network_util.check_if_vlan_interface_exists(self.session, 'vmnet0',
self.cluster).AndReturn(True)
network_util.create_port_group(self.session, 'fa0', 'vmnet0', 3,
self.cluster)
network_util.get_network_with_the_name(self.session, 'fa0', None)
self.mox.ReplayAll()
vif.ensure_vlan_bridge(self.session, self.vif, create_vlan=True)
# FlatDHCP network mode without vlan - network doesn't exist with the host
def test_ensure_vlan_bridge_without_vlan(self):
self.mox.StubOutWithMock(network_util, 'get_network_with_the_name')
self.mox.StubOutWithMock(network_util,
'get_vswitch_for_vlan_interface')
self.mox.StubOutWithMock(network_util,
'check_if_vlan_interface_exists')
self.mox.StubOutWithMock(network_util, 'create_port_group')
network_util.get_network_with_the_name(self.session, 'fa0',
self.cluster).AndReturn(None)
network_util.get_vswitch_for_vlan_interface(self.session, 'vmnet0',
self.cluster).AndReturn('vmnet0')
network_util.check_if_vlan_interface_exists(self.session, 'vmnet0',
self.cluster).AndReturn(True)
network_util.create_port_group(self.session, 'fa0', 'vmnet0', 0,
self.cluster)
network_util.get_network_with_the_name(self.session, 'fa0', None)
self.mox.ReplayAll()
vif.ensure_vlan_bridge(self.session, self.vif, create_vlan=False)
# FlatDHCP network mode without vlan - network exists with the host
# Get vswitch and check vlan interface should not be called
def test_ensure_vlan_bridge_with_network(self):
self.mox.StubOutWithMock(network_util, 'get_network_with_the_name')
self.mox.StubOutWithMock(network_util,
'get_vswitch_for_vlan_interface')
self.mox.StubOutWithMock(network_util,
'check_if_vlan_interface_exists')
self.mox.StubOutWithMock(network_util, 'create_port_group')
vm_network = {'name': 'VM Network', 'type': 'Network'}
network_util.get_network_with_the_name(self.session, 'fa0',
self.cluster).AndReturn(vm_network)
self.mox.ReplayAll()
vif.ensure_vlan_bridge(self.session, self.vif, create_vlan=False)
# Flat network mode with DVS
def test_ensure_vlan_bridge_with_existing_dvs(self):
network_ref = {'dvpg': 'dvportgroup-2062',
'type': 'DistributedVirtualPortgroup'}
self.mox.StubOutWithMock(network_util, 'get_network_with_the_name')
self.mox.StubOutWithMock(network_util,
'get_vswitch_for_vlan_interface')
self.mox.StubOutWithMock(network_util,
'check_if_vlan_interface_exists')
self.mox.StubOutWithMock(network_util, 'create_port_group')
network_util.get_network_with_the_name(self.session, 'fa0',
self.cluster).AndReturn(network_ref)
self.mox.ReplayAll()
ref = vif.ensure_vlan_bridge(self.session,
self.vif,
create_vlan=False)
self.assertThat(ref, matchers.DictMatches(network_ref))
def test_get_network_ref_neutron(self):
self.mox.StubOutWithMock(vif, 'get_neutron_network')
vif.get_neutron_network(self.session, 'fa0', self.cluster, self.vif)
self.mox.ReplayAll()
vif.get_network_ref(self.session, self.cluster, self.vif, True)
def test_get_network_ref_flat_dhcp(self):
self.mox.StubOutWithMock(vif, 'ensure_vlan_bridge')
vif.ensure_vlan_bridge(self.session, self.vif, cluster=self.cluster,
create_vlan=False)
self.mox.ReplayAll()
vif.get_network_ref(self.session, self.cluster, self.vif, False)
def test_get_network_ref_bridge(self):
self.mox.StubOutWithMock(vif, 'ensure_vlan_bridge')
vif.ensure_vlan_bridge(self.session, self.vif, cluster=self.cluster,
create_vlan=True)
self.mox.ReplayAll()
network = network_model.Network(id=0,
bridge='fa0',
label='fake',
vlan=3,
bridge_interface='eth0',
injected=True,
should_create_vlan=True)
self.vif = network_model.NetworkInfo([
network_model.VIF(id=None,
address='DE:AD:BE:EF:00:00',
network=network,
type=None,
devname=None,
ovs_interfaceid=None,
rxtx_cap=3)
])[0]
vif.get_network_ref(self.session, self.cluster, self.vif, False)
def test_get_network_ref_bridge_from_opaque(self):
opaque_networks = [{'opaqueNetworkId': 'bridge_id',
'opaqueNetworkName': 'name',
'opaqueNetworkType': 'OpaqueNetwork'}]
network_ref = vif._get_network_ref_from_opaque(opaque_networks,
'integration_bridge', 'bridge_id')
self.assertEqual('bridge_id', network_ref['network-id'])
def test_get_network_ref_multiple_bridges_from_opaque(self):
opaque_networks = [{'opaqueNetworkId': 'bridge_id1',
'opaqueNetworkName': 'name1',
'opaqueNetworkType': 'OpaqueNetwork'},
{'opaqueNetworkId': 'bridge_id2',
'opaqueNetworkName': 'name2',
'opaqueNetworkType': 'OpaqueNetwork'}]
network_ref = vif._get_network_ref_from_opaque(opaque_networks,
'integration_bridge', 'bridge_id2')
self.assertEqual('bridge_id2', network_ref['network-id'])
def test_get_network_ref_integration(self):
opaque_networks = [{'opaqueNetworkId': 'integration_bridge',
'opaqueNetworkName': 'name',
'opaqueNetworkType': 'OpaqueNetwork'}]
network_ref = vif._get_network_ref_from_opaque(opaque_networks,
'integration_bridge', 'bridge_id')
self.assertEqual('integration_bridge', network_ref['network-id'])
def test_get_network_ref_bridge_none(self):
opaque_networks = [{'opaqueNetworkId': 'bridge_id1',
'opaqueNetworkName': 'name1',
'opaqueNetworkType': 'OpaqueNetwork'},
{'opaqueNetworkId': 'bridge_id2',
'opaqueNetworkName': 'name2',
'opaqueNetworkType': 'OpaqueNetwork'}]
network_ref = vif._get_network_ref_from_opaque(opaque_networks,
'integration_bridge', 'bridge_id')
self.assertIsNone(network_ref)
def test_get_network_ref_integration_multiple(self):
opaque_networks = [{'opaqueNetworkId': 'bridge_id1',
'opaqueNetworkName': 'name1',
'opaqueNetworkType': 'OpaqueNetwork'},
{'opaqueNetworkId': 'integration_bridge',
'opaqueNetworkName': 'name2',
'opaqueNetworkType': 'OpaqueNetwork'}]
network_ref = vif._get_network_ref_from_opaque(opaque_networks,
'integration_bridge', 'bridge_id')
self.assertIsNone(network_ref)
def test_get_neutron_network(self):
self.mox.StubOutWithMock(vm_util, 'get_host_ref')
self.mox.StubOutWithMock(self.session, '_call_method')
self.mox.StubOutWithMock(vif, '_get_network_ref_from_opaque')
vm_util.get_host_ref(self.session,
self.cluster).AndReturn('fake-host')
opaque = fake.DataObject()
opaque.HostOpaqueNetworkInfo = ['fake-network-info']
self.session._call_method(vutil, "get_object_property",
'fake-host', 'config.network.opaqueNetwork').AndReturn(opaque)
vif._get_network_ref_from_opaque(opaque.HostOpaqueNetworkInfo,
CONF.vmware.integration_bridge,
self.vif['network']['id']).AndReturn('fake-network-ref')
self.mox.ReplayAll()
network_ref = vif.get_neutron_network(self.session,
self.vif['network']['id'],
self.cluster,
self.vif)
self.assertEqual(network_ref, 'fake-network-ref')
def test_get_neutron_network_opaque_network_not_found(self):
self.mox.StubOutWithMock(vm_util, 'get_host_ref')
self.mox.StubOutWithMock(self.session, '_call_method')
self.mox.StubOutWithMock(vif, '_get_network_ref_from_opaque')
vm_util.get_host_ref(self.session,
self.cluster).AndReturn('fake-host')
opaque = fake.DataObject()
opaque.HostOpaqueNetworkInfo = ['fake-network-info']
self.session._call_method(vutil, "get_object_property",
'fake-host', 'config.network.opaqueNetwork').AndReturn(opaque)
vif._get_network_ref_from_opaque(opaque.HostOpaqueNetworkInfo,
CONF.vmware.integration_bridge,
self.vif['network']['id']).AndReturn(None)
self.mox.ReplayAll()
self.assertRaises(exception.NetworkNotFoundForBridge,
vif.get_neutron_network, self.session,
self.vif['network']['id'], self.cluster, self.vif)
def test_get_neutron_network_bridge_network_not_found(self):
self.mox.StubOutWithMock(vm_util, 'get_host_ref')
self.mox.StubOutWithMock(self.session, '_call_method')
self.mox.StubOutWithMock(network_util, 'get_network_with_the_name')
vm_util.get_host_ref(self.session,
self.cluster).AndReturn('fake-host')
opaque = fake.DataObject()
opaque.HostOpaqueNetworkInfo = ['fake-network-info']
self.session._call_method(vutil, "get_object_property",
'fake-host', 'config.network.opaqueNetwork').AndReturn(None)
network_util.get_network_with_the_name(self.session, 0,
self.cluster).AndReturn(None)
self.mox.ReplayAll()
self.assertRaises(exception.NetworkNotFoundForBridge,
vif.get_neutron_network, self.session,
self.vif['network']['id'], self.cluster, self.vif)
def test_create_port_group_already_exists(self):
def fake_call_method(module, method, *args, **kwargs):
if method == 'AddPortGroup':
raise vexc.AlreadyExistsException()
with test.nested(
mock.patch.object(vm_util, 'get_add_vswitch_port_group_spec'),
mock.patch.object(vm_util, 'get_host_ref'),
mock.patch.object(self.session, '_call_method',
fake_call_method)
) as (_add_vswitch, _get_host, _call_method):
network_util.create_port_group(self.session, 'pg_name',
'vswitch_name', vlan_id=0,
cluster=None)
def test_create_port_group_exception(self):
def fake_call_method(module, method, *args, **kwargs):
if method == 'AddPortGroup':
raise vexc.VMwareDriverException()
with test.nested(
mock.patch.object(vm_util, 'get_add_vswitch_port_group_spec'),
mock.patch.object(vm_util, 'get_host_ref'),
mock.patch.object(self.session, '_call_method',
fake_call_method)
) as (_add_vswitch, _get_host, _call_method):
self.assertRaises(vexc.VMwareDriverException,
network_util.create_port_group,
self.session, 'pg_name',
'vswitch_name', vlan_id=0,
cluster=None)
def test_get_neutron_network_invalid_property(self):
def fake_call_method(module, method, *args, **kwargs):
if method == 'get_object_property':
raise vexc.InvalidPropertyException()
with test.nested(
mock.patch.object(vm_util, 'get_host_ref'),
mock.patch.object(self.session, '_call_method',
fake_call_method),
mock.patch.object(network_util, 'get_network_with_the_name')
) as (_get_host, _call_method, _get_name):
vif.get_neutron_network(self.session, 'network_name',
'cluster', self.vif)
def test_get_vif_info_none(self):
vif_info = vif.get_vif_info('fake_session', 'fake_cluster',
'is_neutron', 'fake_model', None)
self.assertEqual([], vif_info)
def test_get_vif_info_empty_list(self):
vif_info = vif.get_vif_info('fake_session', 'fake_cluster',
'is_neutron', 'fake_model', [])
self.assertEqual([], vif_info)
@mock.patch.object(vif, 'get_network_ref', return_value='fake_ref')
def test_get_vif_info(self, mock_get_network_ref):
network_info = utils.get_test_network_info()
vif_info = vif.get_vif_info('fake_session', 'fake_cluster',
'is_neutron', 'fake_model', network_info)
expected = [{'iface_id': 'vif-xxx-yyy-zzz',
'mac_address': 'fake',
'network_name': 'fake',
'network_ref': 'fake_ref',
'vif_model': 'fake_model'}]
self.assertEqual(expected, vif_info)
| raildo/nova | nova/tests/unit/virt/vmwareapi/test_vif.py | Python | apache-2.0 | 16,646 |
from build.management.commands.base_build import Command as BaseBuild
from build.management.commands.build_homology_models_zip import Command as UploadModel
from django.db.models import Q
from django.conf import settings
from protein.models import Protein, ProteinConformation, ProteinAnomaly, ProteinState, ProteinSegment
from residue.models import Residue
from residue.functions import dgn, ggn
from structure.models import *
from structure.functions import HSExposureCB, PdbStateIdentifier, update_template_source, StructureSeqNumOverwrite
from common.alignment import AlignedReferenceTemplate, GProteinAlignment
from common.definitions import *
from common.models import WebLink
from signprot.models import SignprotComplex
import structure.structural_superposition as sp
import structure.assign_generic_numbers_gpcr as as_gn
import structure.homology_models_tests as tests
from structure.signprot_modeling import SignprotModeling
from structure.homology_modeling_functions import GPCRDBParsingPDB, ImportHomologyModel, Remodeling
import Bio.PDB as PDB
from modeller import *
from modeller.automodel import *
from collections import OrderedDict
import os
import shlex
import logging
import pprint
from io import StringIO, BytesIO
import sys
import re
import zipfile
import shutil
import math
from copy import deepcopy
from datetime import datetime, date
import yaml
import traceback
import subprocess
startTime = datetime.now()
class Command(BaseBuild):
help = 'Build automated chimeric GPCR homology models'
models_with_knots = []
def add_arguments(self, parser):
super(Command, self).add_arguments(parser=parser)
parser.add_argument('--path', help='Path for zipped model files to check', default=False, type=str)
def handle(self, *args, **options):
self.remove_models_with_knots()
return 0
if options['path']:
self.path = options['path']
else:
self.path = './structure/complex_models_zip/'
self.c1, self.c2 = 0,0
self.file_list = [i for i in os.listdir(self.path) if i.endswith('zip')]
# self.file_list = ['ClassA_mc5r_human-gnai2_human_6D9H_2019-03-22_GPCRdb.zip']
self.processors = options['proc']
self.prepare_input(options['proc'], self.file_list)
# print(self.models_with_knots)
# print(len(self.file_list), len(self.models_with_knots), str(len(self.models_with_knots)/len(self.file_list)*100)+'%')
datetime.now() - startTime
def main_func(self, positions, iteration, count, lock):
processor_id = round(self.processors*positions[0]/len(self.file_list))+1
i = 0
while count.value<len(self.file_list):
i += 1
with lock:
zipname = self.file_list[count.value]
count.value +=1
modelname = zipname.split('.')[0]
zip_ref = zipfile.ZipFile(self.path+zipname, 'r')
zip_ref.extractall(self.path+modelname+'/')
zip_ref.close()
p = PDB.PDBParser()
classname, receptor, species_signprot, species, main_temp, date, placeholder = modelname.split('_')
receptor_obj = Protein.objects.get(entry_name=receptor+'_'+species_signprot.split('-')[0])
signprot_obj = Protein.objects.get(entry_name=species_signprot.split('-')[1]+'_'+species)
model = p.get_structure('model', self.path+modelname+'/'+modelname+'.pdb')
hse = HSExposureCB(model, radius=9.5, check_chain_breaks=True, check_knots=True, receptor=receptor_obj, signprot=signprot_obj, restrict_to_chain=['A','R'])
# print(zipname)
# print(hse.remodel_resis)
if len(hse.remodel_resis)>0:
print(zipname)
# self.models_with_knots.append(zipname)
# print(self.models_with_knots)
shutil.rmtree(self.path+modelname)
def remove_models_with_knots(self):
with open('./complex_knots_HE_hdhe.txt', 'r') as f:
zips = f.readlines()
for z in zips:
z = z[:-1]
try:
shutil.move('./structure/complex_models_zip/'+z, './structure/models_with_knots/'+z)
except:
pass
| cmunk/protwis | structure/management/commands/check_knots.py | Python | apache-2.0 | 3,856 |
#!/usr/bin/env python
# -*- coding: utf-8 -*-
###############################################################################
# Copyright 2014 Kitware Inc.
#
# Licensed under the Apache License, Version 2.0 ( the "License" );
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
###############################################################################
from ..rest import Resource
from ..describe import Description
from girder.api import access
from girder.constants import TokenScope
class Token(Resource):
"""API Endpoint for non-user tokens in the system."""
def __init__(self):
self.resourceName = 'token'
self.route('DELETE', ('session',), self.deleteSession)
self.route('GET', ('session',), self.getSession)
self.route('GET', ('current',), self.currentSession)
@access.public
def currentSession(self, params):
token = self.getCurrentToken()
return token
currentSession.description = (
Description('Retrieve the current session information.')
.responseClass('Token'))
@access.public
def getSession(self, params):
"""
Create an anonymous session. Sends an auth cookie in the response on
success.
"""
token = self.getCurrentToken()
# Only create and send new cookie if token isn't valid or will expire
# soon
if not token:
token = self.sendAuthTokenCookie(
None, scope=TokenScope.ANONYMOUS_SESSION)
return {
'token': token['_id'],
'expires': token['expires']
}
getSession.description = (
Description('Get an anonymous session token for the system.')
.notes('If you are logged in, this will return a token associated '
'with that login.')
.responseClass('Token'))
@access.token
def deleteSession(self, params):
token = self.getCurrentToken()
if token:
self.model('token').remove(token)
self.deleteAuthTokenCookie()
return {'message': 'Session deleted.'}
deleteSession.description = (
Description('Remove a session from the system.')
.responseClass('Token')
.notes('Attempts to delete your authentication cookie.'))
| chrismattmann/girder | girder/api/v1/token.py | Python | apache-2.0 | 2,716 |
from __future__ import print_function
import sys
sys.path.insert(1,"../../")
import h2o
from tests import pyunit_utils
from h2o.transforms.preprocessing import H2OScaler
from h2o.estimators.random_forest import H2ORandomForestEstimator
from sklearn.pipeline import Pipeline
from sklearn.model_selection import RandomizedSearchCV
from h2o.cross_validation import H2OKFold
from h2o.model.regression import h2o_r2_score
from sklearn.metrics.scorer import make_scorer
from scipy.stats import randint
def scale_svd_rf_pipe():
from h2o.transforms.decomposition import H2OSVD
print("Importing USArrests.csv data...")
arrests = h2o.upload_file(pyunit_utils.locate("smalldata/pca_test/USArrests.csv"))
# build transformation pipeline using sklearn's Pipeline and H2OSVD
pipe = Pipeline([
("standardize", H2OScaler()),
("svd", H2OSVD()),
("rf", H2ORandomForestEstimator())
])
params = {"standardize__center": [True, False],
"standardize__scale": [True, False],
"svd__nv": [2, 3],
"rf__ntrees": randint(50,60),
"rf__max_depth": randint(4,8),
"rf__min_rows": randint(5,10),
"svd__transform": ["none", "standardize"],
}
custom_cv = H2OKFold(arrests, n_folds=5, seed=42)
random_search = RandomizedSearchCV(pipe,
params,
n_iter=5,
scoring=make_scorer(h2o_r2_score),
cv=custom_cv,
random_state=42,
n_jobs=1)
random_search.fit(arrests[1:],arrests[0])
print(random_search.best_estimator_)
def scale_svd_rf_pipe_new_import():
from h2o.estimators.svd import H2OSingularValueDecompositionEstimator
print("Importing USArrests.csv data...")
arrests = h2o.upload_file(pyunit_utils.locate("smalldata/pca_test/USArrests.csv"))
print("Compare with SVD")
# build transformation pipeline using sklearn's Pipeline and H2OSingularValueDecompositionEstimator
pipe = Pipeline([
("standardize", H2OScaler()),
# H2OSingularValueDecompositionEstimator() call will fail, you have to call init_for_pipeline method
("svd", H2OSingularValueDecompositionEstimator().init_for_pipeline()),
("rf", H2ORandomForestEstimator())
])
params = {"standardize__center": [True, False],
"standardize__scale": [True, False],
"svd__nv": [2, 3],
"rf__ntrees": randint(50,60),
"rf__max_depth": randint(4,8),
"rf__min_rows": randint(5,10),
"svd__transform": ["none", "standardize"],
}
custom_cv = H2OKFold(arrests, n_folds=5, seed=42)
random_search = RandomizedSearchCV(pipe,
params,
n_iter=5,
scoring=make_scorer(h2o_r2_score),
cv=custom_cv,
random_state=42,
n_jobs=1)
random_search.fit(arrests[1:], arrests[0])
print(random_search.best_estimator_)
if __name__ == "__main__":
pyunit_utils.standalone_test(scale_svd_rf_pipe)
pyunit_utils.standalone_test(scale_svd_rf_pipe_new_import)
else:
scale_svd_rf_pipe()
scale_svd_rf_pipe_new_import()
| h2oai/h2o-3 | h2o-py/tests/testdir_scikit_grid/pyunit_scal_svd_rf_grid.py | Python | apache-2.0 | 3,661 |
#!/usr/bin/env python
"""
main.py -- Udacity conference server-side Python App Engine
HTTP controller handlers for memcache & task queue access
$Id$
created by wesc on 2014 may 24
"""
__author__ = '[email protected] (Wesley Chun)'
import webapp2
from google.appengine.api import app_identity
from google.appengine.api import mail
from conference import ConferenceApi
class SetAnnouncementHandler(webapp2.RequestHandler):
def get(self):
"""Set Announcement in Memcache."""
ConferenceApi._cacheAnnouncement()
self.response.set_status(204)
class SendConfirmationEmailHandler(webapp2.RequestHandler):
def post(self):
"""Send email confirming Conference creation."""
mail.send_mail(
'noreply@%s.appspotmail.com' % (
app_identity.get_application_id()), # from
self.request.get('email'), # to
'You created a new Conference!', # subj
'Hi, you have created a following ' # body
'conference:\r\n\r\n%s' % self.request.get(
'conferenceInfo')
)
class SetFeaturedSpeakerHandler(webapp2.RequestHandler):
def post(self):
"""Set Featured Speaker in Memcache."""
websafeConferenceKey = self.request.get('websafeConferenceKey')
speaker = self.request.get('speaker')
ConferenceApi.setFeaturedSpeaker(websafeConferenceKey, speaker)
app = webapp2.WSGIApplication([
('/crons/set_announcement', SetAnnouncementHandler),
('/tasks/send_confirmation_email', SendConfirmationEmailHandler),
('/tasks/set_featured_speaker', SetFeaturedSpeakerHandler),
], debug=True)
| mrrobeson/Project-4---Conference-App | main.py | Python | apache-2.0 | 1,695 |
#!/usr/bin/env python
#-------------------------------------------------------------------------
# Copyright (c) Microsoft. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
#--------------------------------------------------------------------------
from distutils.core import setup
setup(name='ptvsd',
version='2.2.0b1',
description='Python Tools for Visual Studio remote debugging server',
license='Apache License 2.0',
author='Microsoft Corporation',
author_email='[email protected]',
url='https://aka.ms/ptvs',
classifiers=[
'Development Status :: 4 - Beta',
'Programming Language :: Python',
'Programming Language :: Python :: 2',
'Programming Language :: Python :: 3',
'License :: OSI Approved :: Apache Software License'],
packages=['ptvsd']
)
| juanyaw/PTVS | Python/Product/PythonTools/ptvsd/setup.py | Python | apache-2.0 | 1,400 |
# Copyright 2017-present Open Networking Foundation
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
from protos import dynamicload_pb2
from protos import dynamicload_pb2_grpc
from dynamicbuild import DynamicBuilder
from apistats import REQUEST_COUNT, track_request_time
from authhelper import XOSAuthHelperMixin
from decorators import translate_exceptions, require_authentication, check_db_connection
import grpc
import semver
from xosconfig import Config
from multistructlog import create_logger
log = create_logger(Config().get("logging"))
class DynamicLoadService(dynamicload_pb2_grpc.dynamicloadServicer, XOSAuthHelperMixin):
def __init__(self, thread_pool, server):
self.thread_pool = thread_pool
self.server = server
self.django_apps = None
self.django_apps_by_name = {}
XOSAuthHelperMixin.__init__(self)
def stop(self):
pass
def set_django_apps(self, django_apps):
""" Allows the creator of DynamicLoadService to pass in a pointer to django's app list once django has been
installed. This is optional. We don't import django.apps directly, as DynamicLoadService must be able
to run even if django is broken due to modeling issues.
"""
self.django_apps = django_apps
# Build up some dictionaries used by the API handlers. We can build these once at initialization time because
# when apps are onboarded, the core is always restarted.
self.django_apps_by_name = {}
self.django_app_models = {}
if self.django_apps:
for app in self.django_apps.get_app_configs():
self.django_apps_by_name[app.name] = app
# Build up a dictionary of all non-decl models.
django_models = {}
for (k, v) in app.models.items():
if not k.endswith("_decl"):
django_models[k] = v
self.django_app_models[app.name] = django_models
def get_core_version(self):
try:
core_version = open("/opt/xos/VERSION").readline().strip()
except Exception:
core_version = "unknown"
return core_version
@track_request_time("DynamicLoad", "LoadModels")
@translate_exceptions("DynamicLoad", "LoadModels")
@check_db_connection
@require_authentication
def LoadModels(self, request, context):
try:
core_version = self.get_core_version()
requested_core_version = request.core_version
log.info("Loading service models",
service=request.name,
service_version=request.version,
requested_core_version=requested_core_version
)
if not requested_core_version:
requested_core_version = ">=2.2.1"
if "~" in requested_core_version:
[min_requested, max_requested] = requested_core_version.split("~")
match_min_version = semver.match(core_version, min_requested.strip())
match_max_version = semver.match(core_version, max_requested.strip())
if not match_min_version or not match_max_version:
log.error("Not loading service because of mismatching versions",
service=request.name,
core_version=core_version,
requested_min_core_version=min_requested,
requested_max_core_version=max_requested
)
context.set_code(grpc.StatusCode.INVALID_ARGUMENT)
msg = "Service %s is requesting core version between %s and %s but actual version is %s" % (
request.name,
min_requested,
max_requested,
core_version
)
context.set_details(msg)
raise Exception(msg)
else:
match_version = semver.match(core_version, requested_core_version.strip())
if not match_version:
log.error("Not loading service because of mismatching versions", service=request.name,
core_version=core_version, requested_core_version=requested_core_version)
context.set_code(grpc.StatusCode.INVALID_ARGUMENT)
msg = "Service %s is requesting core version %s but actual version is %s" % (
request.name, requested_core_version, core_version)
context.set_details(msg)
raise Exception(msg)
builder = DynamicBuilder()
result = builder.handle_loadmodels_request(request)
if result == builder.SUCCESS:
self.server.delayed_shutdown(5)
response = dynamicload_pb2.LoadModelsReply()
response.status = result
REQUEST_COUNT.labels(
"xos-core", "DynamicLoad", "LoadModels", grpc.StatusCode.OK
).inc()
return response
except Exception as e:
import traceback
traceback.print_exc()
REQUEST_COUNT.labels(
"xos-core", "DynamicLoad", "LoadModels", grpc.StatusCode.INTERNAL
).inc()
raise e
def map_error_code(self, status, context):
""" Map the DynamicLoad status into an appropriate gRPC status code
and include an error description if appropriate.
Chameleon supports limited mapping to http status codes.
Picked a best-fit:
OK = 200
INVALID_ARGUMENT = 400
ALREADY_EXISTS = 409
"""
code_names = {
DynamicBuilder.SUCCESS: "SUCCESS",
DynamicBuilder.SUCCESS_NOTHING_CHANGED: "SUCCESS_NOTHING_CHANGED",
DynamicBuilder.ERROR: "ERROR",
DynamicBuilder.ERROR_LIVE_MODELS: "ERROR_LIVE_MODELS",
DynamicBuilder.ERROR_DELETION_IN_PROGRESS: "ERROR_DELETION_IN_PROGRESS",
DynamicBuilder.TRYAGAIN: "TRYAGAIN"}
code_map = {
DynamicBuilder.SUCCESS: grpc.StatusCode.OK,
DynamicBuilder.SUCCESS_NOTHING_CHANGED: grpc.StatusCode.OK,
DynamicBuilder.ERROR: grpc.StatusCode.INVALID_ARGUMENT,
DynamicBuilder.ERROR_LIVE_MODELS: grpc.StatusCode.ALREADY_EXISTS,
DynamicBuilder.ERROR_DELETION_IN_PROGRESS: grpc.StatusCode.ALREADY_EXISTS,
DynamicBuilder.TRYAGAIN: grpc.StatusCode.OK}
code = code_map.get(status, DynamicBuilder.ERROR)
context.set_code(code)
if code != grpc.StatusCode.OK:
# In case of error, send helpful text back to the caller
context.set_details(code_names.get(status, "UNKNOWN"))
@track_request_time("DynamicLoad", "UnloadModels")
@translate_exceptions("DynamicLoad", "UnloadModels")
@check_db_connection
@require_authentication
def UnloadModels(self, request, context):
try:
builder = DynamicBuilder()
result = builder.handle_unloadmodels_request(request,
self.django_app_models.get("services." + request.name, {}))
if result == builder.SUCCESS:
self.server.delayed_shutdown(5)
self.map_error_code(result, context)
response = dynamicload_pb2.LoadModelsReply()
response.status = result
REQUEST_COUNT.labels(
"xos-core", "DynamicLoad", "UnloadModels", grpc.StatusCode.OK
).inc()
return response
except Exception as e:
import traceback
traceback.print_exc()
REQUEST_COUNT.labels(
"xos-core", "DynamicLoad", "UnloadModels", grpc.StatusCode.INTERNAL
).inc()
raise e
@track_request_time("DynamicLoad", "GetLoadStatus")
@translate_exceptions("DynamicLoad", "GetLoadStatus")
@check_db_connection
@require_authentication
def GetLoadStatus(self, request, context):
try:
builder = DynamicBuilder()
manifests = builder.get_manifests()
response = dynamicload_pb2.LoadStatusReply()
response.model_status = self.server.model_status
response.model_output = self.server.model_output
for manifest in manifests:
item = response.services.add()
item.name = manifest["name"]
item.version = manifest["version"]
item.state = manifest.get("state", "unspecified")
if item.state == "load":
django_app = self.django_apps_by_name.get("services." + item.name)
if django_app:
item.state = "present"
# TODO: Might be useful to return a list of models as well
# the core is always onboarded, so doesn't have an explicit manifest
item = response.services.add()
item.name = "core"
item.version = self.get_core_version()
if "core" in self.django_apps_by_name:
item.state = "present"
else:
item.state = "load"
REQUEST_COUNT.labels(
"xos-core", "DynamicLoad", "GetLoadStatus", grpc.StatusCode.OK
).inc()
return response
except Exception as e:
import traceback
traceback.print_exc()
REQUEST_COUNT.labels(
"xos-core", "DynamicLoad", "GetLoadStatus", grpc.StatusCode.INTERNAL
).inc()
raise e
@track_request_time("DynamicLoad", "GetConvenienceMethods")
@translate_exceptions("DynamicLoad", "GetConvenienceMethods")
@check_db_connection
@require_authentication
def GetConvenienceMethods(self, request, context):
# self.authenticate(context, required=True)
try:
builder = DynamicBuilder()
manifests = builder.get_manifests()
response = dynamicload_pb2.ListConvenienceMethodsReply()
for manifest in manifests:
for cm in manifest["convenience_methods"]:
item = response.convenience_methods.add()
item.filename = cm["filename"]
item.contents = open(cm["path"]).read()
REQUEST_COUNT.labels(
"xos-core", "DynamicLoad", "GetConvenienceMethods", grpc.StatusCode.OK
).inc()
return response
except Exception as e:
import traceback
traceback.print_exc()
REQUEST_COUNT.labels(
"xos-core",
"DynamicLoad",
"GetConvenienceMethods",
grpc.StatusCode.INTERNAL,
).inc()
raise e
| opencord/xos | xos/coreapi/xos_dynamicload_api.py | Python | apache-2.0 | 11,546 |
import uuid
from keystoneclient.v3 import policies
from tests.v3 import utils
class PolicyTests(utils.TestCase, utils.CrudTests):
def setUp(self):
super(PolicyTests, self).setUp()
self.additionalSetUp()
self.key = 'policy'
self.collection_key = 'policies'
self.model = policies.Policy
self.manager = self.client.policies
def new_ref(self, **kwargs):
kwargs = super(PolicyTests, self).new_ref(**kwargs)
kwargs.setdefault('type', uuid.uuid4().hex)
kwargs.setdefault('blob', uuid.uuid4().hex)
return kwargs
| tylertian/Openstack | openstack F/python-keystoneclient/tests/v3/test_policies.py | Python | apache-2.0 | 596 |
#!/usr/bin/env python
#page 39
import shelve
db = shelve.open('../db/people-shelve')
for key in db:
print(key, '=>\n', db[key])
print(db['kouer']['name'])
db.close()
| lichengshuang/createvhost | python/others/Preview/dump_db_shelve.py | Python | apache-2.0 | 171 |
from hazelcast.serialization.bits import *
from hazelcast.protocol.client_message import ClientMessage
from hazelcast.protocol.custom_codec import *
from hazelcast.util import ImmutableLazyDataList
from hazelcast.protocol.codec.map_message_type import *
REQUEST_TYPE = MAP_EXECUTEONKEYS
RESPONSE_TYPE = 117
RETRYABLE = False
def calculate_size(name, entry_processor, keys):
""" Calculates the request payload size"""
data_size = 0
data_size += calculate_size_str(name)
data_size += calculate_size_data(entry_processor)
data_size += INT_SIZE_IN_BYTES
for keys_item in keys:
data_size += calculate_size_data(keys_item)
return data_size
def encode_request(name, entry_processor, keys):
""" Encode request into client_message"""
client_message = ClientMessage(payload_size=calculate_size(name, entry_processor, keys))
client_message.set_message_type(REQUEST_TYPE)
client_message.set_retryable(RETRYABLE)
client_message.append_str(name)
client_message.append_data(entry_processor)
client_message.append_int(len(keys))
for keys_item in keys:
client_message.append_data(keys_item)
client_message.update_frame_length()
return client_message
def decode_response(client_message, to_object=None):
""" Decode response from client message"""
parameters = dict(response=None)
response_size = client_message.read_int()
response = []
for response_index in xrange(0, response_size):
response_item = (client_message.read_data(), client_message.read_data())
response.append(response_item)
parameters['response'] = ImmutableLazyDataList(response, to_object)
return parameters
| cangencer/hazelcast-python-client | hazelcast/protocol/codec/map_execute_on_keys_codec.py | Python | apache-2.0 | 1,694 |
from warpnet_client import *
from warpnet_common_params import *
from warpnet_experiment_structs import *
from twisted.internet import reactor
from datetime import *
import time
minTime = 10
pktLen = 1412
pktPeriod = 2000
mod_hdr = 2
mod_payload = 2
txGains = [30, 45, 60];
class ScriptMaster:
def startup(self):
er_log = DataLogger('twoNode_PER_Test_v0.m', flushTime=0)
er_log.log("%%WARPnet PER Test Example - %s\r\n" % datetime.now())
registerWithServer()
nodes = dict()
#WARP Nodes
createNode(nodes, Node(0, NODE_PCAP))
createNode(nodes, Node(1, NODE_PCAP))
connectToServer(nodes)
controlStruct = ControlStruct()
nodes[0].addStruct('controlStruct', controlStruct)
nodes[1].addStruct('controlStruct', controlStruct)
cmdStructStart = CommandStruct(COMMANDID_STARTTRIAL, 0)
nodes[0].addStruct('cmdStructStart', cmdStructStart)
cmdStructStop = CommandStruct(COMMANDID_STOPTRIAL, 0)
nodes[0].addStruct('cmdStructStop', cmdStructStop)
cmdStructResetPER = CommandStruct(COMMANDID_RESET_PER, 0)
nodes[0].addStruct('cmdStructResetPER', cmdStructResetPER)
nodes[1].addStruct('cmdStructResetPER', cmdStructResetPER)
perStruct0 = ObservePERStruct()
perStruct1 = ObservePERStruct()
nodes[0].addStruct('perStruct', perStruct0)
nodes[1].addStruct('perStruct', perStruct1)
sendRegistrations(nodes)
controlStruct.packetGeneratorPeriod = pktPeriod
controlStruct.packetGeneratorLength = pktLen
controlStruct.channel = 9
controlStruct.txPower = 63
controlStruct.modOrderHeader = mod_hdr
controlStruct.modOrderPayload = mod_payload
nodes[0].sendToNode('controlStruct')
nodes[1].sendToNode('controlStruct')
nodes[0].sendToNode('cmdStructResetPER')
nodes[1].sendToNode('cmdStructResetPER')
#Experiment loop
for ii, txGain in enumerate(txGains):
print("Starting trial %d with TxGain %d at %s" % (ii, txGain, datetime.now()))
#Stop any traffic that might be running
nodes[0].sendToNode('cmdStructStop')
#Reset the PER counters at all nodes
nodes[0].sendToNode('cmdStructResetPER')
nodes[1].sendToNode('cmdStructResetPER')
controlStruct.txPower = txGain
nodes[0].sendToNode('controlStruct')
#Let things settle
time.sleep(0.25)
#Start the trial
nodes[0].sendToNode('cmdStructStart')
#Run until minTime elapses
time.sleep(minTime)
nodes[0].sendToNode('cmdStructStop')
#Give the nodes and server time to process any final structs
time.sleep(1)
#Request 3 PER struts from each node, verifying the response matches this request
perStruct0.reqNum = 0
perStruct1.reqNum = 0
perStruct0.reqType = 0
perStruct1.reqType = 0
nodes[0].sendToNode('perStruct')
nodes[1].sendToNode('perStruct')
if (perStruct0.reqNum != 0) or (perStruct1.reqNum != 0) or \
(perStruct0.reqType != 0) or (perStruct1.reqType != 0):
print("BAD STATE! Out-of-order PER Struct Received")
#Record the results
er_log.log("n0_txGain(%d) = %d;\t" % (ii+1, txGain))
er_log.log("n0_txPkts(%d) = %d;\t" % (ii+1, perStruct0.numPkts_tx))
er_log.log("n1_rxPkts_good(%d) = %d;\t" % (ii+1, perStruct1.numPkts_rx_good))
er_log.log("n1_rxPkts_GhBp(%d) = %d;\t" % (ii+1, perStruct1.numPkts_rx_goodHdrBadPyld))
er_log.log("n1_rxPkts_BadHdr(%d) = %d;\r\n" % (ii+1, perStruct1.numPkts_rx_badHdr))
print("############################################")
print("############# Experiment Done! #############")
print("############################################")
reactor.callFromThread(reactor.stop)
sm = ScriptMaster()
stdio.StandardIO(CmdReader())
factory = WARPnetClient(sm.startup);
reactor.connectTCP('localhost', 10101, factory)
reactor.run()
| shailcoolboy/Warp-Trinity | ResearchApps/Measurement/examples/TxPower_vs_PER/TxPower_vs_PER.py | Python | bsd-2-clause | 3,716 |
# -*- coding: utf-8 -*-
"""
Pygments tests with example files
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
:copyright: Copyright 2006-2014 by the Pygments team, see AUTHORS.
:license: BSD, see LICENSE for details.
"""
from __future__ import print_function
import os
import pprint
import difflib
import pickle
from pygments.lexers import get_lexer_for_filename, get_lexer_by_name
from pygments.token import Error
from pygments.util import ClassNotFound
STORE_OUTPUT = False
STATS = {}
TESTDIR = os.path.dirname(__file__)
# generate methods
def test_example_files():
global STATS
STATS = {}
outdir = os.path.join(TESTDIR, 'examplefiles', 'output')
if STORE_OUTPUT and not os.path.isdir(outdir):
os.makedirs(outdir)
for fn in os.listdir(os.path.join(TESTDIR, 'examplefiles')):
if fn.startswith('.') or fn.endswith('#'):
continue
absfn = os.path.join(TESTDIR, 'examplefiles', fn)
if not os.path.isfile(absfn):
continue
print(absfn)
code = open(absfn, 'rb').read()
try:
code = code.decode('utf-8')
except UnicodeError:
code = code.decode('latin1')
lx = None
if '_' in fn:
try:
lx = get_lexer_by_name(fn.split('_')[0])
except ClassNotFound:
pass
if lx is None:
try:
lx = get_lexer_for_filename(absfn, code=code)
except ClassNotFound:
raise AssertionError('file %r has no registered extension, '
'nor is of the form <lexer>_filename '
'for overriding, thus no lexer found.'
% fn)
yield check_lexer, lx, fn
N = 7
stats = list(STATS.items())
stats.sort(key=lambda x: x[1][1])
print('\nExample files that took longest absolute time:')
for fn, t in stats[-N:]:
print('%-30s %6d chars %8.2f ms %7.3f ms/char' % ((fn,) + t))
print()
stats.sort(key=lambda x: x[1][2])
print('\nExample files that took longest relative time:')
for fn, t in stats[-N:]:
print('%-30s %6d chars %8.2f ms %7.3f ms/char' % ((fn,) + t))
def check_lexer(lx, fn):
absfn = os.path.join(TESTDIR, 'examplefiles', fn)
fp = open(absfn, 'rb')
try:
text = fp.read()
finally:
fp.close()
text = text.replace(b'\r\n', b'\n')
text = text.strip(b'\n') + b'\n'
try:
text = text.decode('utf-8')
if text.startswith(u'\ufeff'):
text = text[len(u'\ufeff'):]
except UnicodeError:
text = text.decode('latin1')
ntext = []
tokens = []
import time
t1 = time.time()
for type, val in lx.get_tokens(text):
ntext.append(val)
assert type != Error, \
'lexer %s generated error token for %s: %r at position %d' % \
(lx, absfn, val, len(u''.join(ntext)))
tokens.append((type, val))
t2 = time.time()
STATS[os.path.basename(absfn)] = (len(text),
1000 * (t2 - t1), 1000 * (t2 - t1) / len(text))
if u''.join(ntext) != text:
print('\n'.join(difflib.unified_diff(u''.join(ntext).splitlines(),
text.splitlines())))
raise AssertionError('round trip failed for ' + absfn)
# check output against previous run if enabled
if STORE_OUTPUT:
# no previous output -- store it
outfn = os.path.join(TESTDIR, 'examplefiles', 'output', fn)
if not os.path.isfile(outfn):
fp = open(outfn, 'wb')
try:
pickle.dump(tokens, fp)
finally:
fp.close()
return
# otherwise load it and compare
fp = open(outfn, 'rb')
try:
stored_tokens = pickle.load(fp)
finally:
fp.close()
if stored_tokens != tokens:
f1 = pprint.pformat(stored_tokens)
f2 = pprint.pformat(tokens)
print('\n'.join(difflib.unified_diff(f1.splitlines(),
f2.splitlines())))
assert False, absfn
| spencerlyon2/pygments | tests/test_examplefiles.py | Python | bsd-2-clause | 4,257 |
"""A minimal application used by a number of tests and tutorials. It
is not meant to be actually useful in the real world.
The basic idea of min9 is to have most plugins installed. So it is the least
minimal of this series.
This is also a **usage example** of :ref:`plugin_inheritance` because if
overrides the :mod:`lino_xl.lib.contacts` plugin by its own version
``lino.projects.min9.modlib.contacts`` (which is not included in this
documentation tree for technical reasons, and anyway you should
inspect the source code if you want to go futher).
The package has a unit test suite for testing some of the plugins it uses:
.. autosummary::
:toctree:
tests.test_addresses
tests.test_birth_date
tests.test_min2
tests.test_cal
"""
| lino-framework/book | lino_book/projects/min9/__init__.py | Python | bsd-2-clause | 751 |
from webtest import TestApp
from main import app
app = TestApp(app)
def test_that_fails():
response = app.get('/')
assert response
test_that_fails.nosegae_logservice = True
| brunoripa/NoseGAE | examples/app_with_logging/tests/test.py | Python | bsd-2-clause | 186 |
# coding: utf-8
from hashlib import sha1
import os
from django.conf import settings
from django.contrib.auth.models import User, Group
from django.core.urlresolvers import reverse
from django.core.validators import MaxLengthValidator
from django.db import models
from django.db.models import aggregates
from django.db.models.signals import post_save
from django.shortcuts import get_object_or_404
from django.utils.translation import ugettext_lazy as _
from django.utils import timezone
from django_fsm.db.fields import FSMField, transition, can_proceed
from djangobb_forum.fields import AutoOneToOneField, ExtendedImageField, JSONField
from djangobb_forum.util import smiles, convert_text_to_html
from djangobb_forum import settings as forum_settings
if 'south' in settings.INSTALLED_APPS:
from south.modelsinspector import add_introspection_rules
add_introspection_rules([], ['^djangobb_forum\.fields\.AutoOneToOneField',
'^djangobb_forum\.fields\.JSONField',
'^djangobb_forum\.fields\.ExtendedImageField'])
TZ_CHOICES = [(float(x[0]), x[1]) for x in (
(-12, '-12'), (-11, '-11'), (-10, '-10'), (-9.5, '-09.5'), (-9, '-09'),
(-8.5, '-08.5'), (-8, '-08 PST'), (-7, '-07 MST'), (-6, '-06 CST'),
(-5, '-05 EST'), (-4, '-04 AST'), (-3.5, '-03.5'), (-3, '-03 ADT'),
(-2, '-02'), (-1, '-01'), (0, '00 GMT'), (1, '+01 CET'), (2, '+02'),
(3, '+03'), (3.5, '+03.5'), (4, '+04'), (4.5, '+04.5'), (5, '+05'),
(5.5, '+05.5'), (6, '+06'), (6.5, '+06.5'), (7, '+07'), (8, '+08'),
(9, '+09'), (9.5, '+09.5'), (10, '+10'), (10.5, '+10.5'), (11, '+11'),
(11.5, '+11.5'), (12, '+12'), (13, '+13'), (14, '+14'),
)]
SIGN_CHOICES = (
(1, 'PLUS'),
(-1, 'MINUS'),
)
PRIVACY_CHOICES = (
(0, _(u'Display your e-mail address.')),
(1, _(u'Hide your e-mail address but allow form e-mail.')),
(2, _(u'Hide your e-mail address and disallow form e-mail.')),
)
MARKUP_CHOICES = [('bbcode', 'bbcode')]
try:
import markdown
MARKUP_CHOICES.append(("markdown", "markdown"))
except ImportError:
pass
path = os.path.join(settings.STATIC_ROOT, 'djangobb_forum', 'themes')
if os.path.exists(path):
# fix for collectstatic
THEME_CHOICES = [(theme, theme) for theme in os.listdir(path)
if os.path.isdir(os.path.join(path, theme))]
else:
THEME_CHOICES = []
import logging
logger = logging.getLogger(__name__)
akismet_api = None
from akismet import Akismet, AkismetError
try:
if getattr(settings, 'AKISMET_ENABLED', True):
akismet_api = Akismet(key=forum_settings.AKISMET_API_KEY, blog_url=forum_settings.AKISMET_BLOG_URL, agent=forum_settings.AKISMET_AGENT)
except Exception as e:
logger.error("Error while initializing Akismet", extra={'exception': e})
class Category(models.Model):
name = models.CharField(_('Name'), max_length=80)
groups = models.ManyToManyField(Group, blank=True, null=True, verbose_name=_('Groups'), help_text=_('Only users from these groups can see this category'))
position = models.IntegerField(_('Position'), blank=True, default=0)
class Meta:
ordering = ['position']
verbose_name = _('Category')
verbose_name_plural = _('Categories')
def __unicode__(self):
return self.name
def forum_count(self):
return self.forums.all().count()
@property
def topics(self):
return Topic.objects.filter(forum__category__id=self.id).select_related()
@property
def posts(self):
return Post.objects.filter(topic__forum__category__id=self.id).select_related()
def has_access(self, user):
if user.is_superuser:
return True
if self.groups.exists():
if user.is_authenticated():
if not self.groups.filter(user__pk=user.id).exists():
return False
else:
return False
return True
class Forum(models.Model):
category = models.ForeignKey(Category, related_name='forums', verbose_name=_('Category'))
moderator_only = models.BooleanField(_('New topics by moderators only'), default=False)
name = models.CharField(_('Name'), max_length=80)
position = models.IntegerField(_('Position'), blank=True, default=0)
description = models.TextField(_('Description'), blank=True, default='')
moderators = models.ManyToManyField(User, blank=True, null=True, verbose_name=_('Moderators'))
updated = models.DateTimeField(_('Updated'), auto_now=True)
post_count = models.IntegerField(_('Post count'), blank=True, default=0)
topic_count = models.IntegerField(_('Topic count'), blank=True, default=0)
last_post = models.ForeignKey('Post', related_name='last_forum_post', blank=True, null=True)
class Meta:
ordering = ['position']
verbose_name = _('Forum')
verbose_name_plural = _('Forums')
def __unicode__(self):
return self.name
@models.permalink
def get_absolute_url(self):
return ('djangobb:forum', [self.id])
def get_mobile_url(self):
return reverse('djangobb:mobile_forum', args=[self.id])
@property
def posts(self):
return Post.objects.filter(topic__forum__id=self.id).select_related()
def set_last_post(self):
try:
self.last_post = Topic.objects.filter(forum=self).latest().last_post
except Topic.DoesNotExist:
self.last_post = None
def set_counts(self):
self.topic_count = Topic.objects.filter(forum=self).count()
self.post_count = Post.objects.filter(topic__forum=self).count()
class Topic(models.Model):
forum = models.ForeignKey(Forum, related_name='topics', verbose_name=_('Forum'))
name = models.CharField(_('Subject'), max_length=255)
created = models.DateTimeField(_('Created'), auto_now_add=True)
updated = models.DateTimeField(_('Updated'), null=True)
user = models.ForeignKey(User, verbose_name=_('User'))
views = models.IntegerField(_('Views count'), blank=True, default=0)
sticky = models.BooleanField(_('Sticky'), blank=True, default=False)
closed = models.BooleanField(_('Closed'), blank=True, default=False)
subscribers = models.ManyToManyField(User, related_name='subscriptions', verbose_name=_('Subscribers'), blank=True)
post_count = models.IntegerField(_('Post count'), blank=True, default=0)
last_post = models.ForeignKey('Post', related_name='last_topic_post', blank=True, null=True)
class Meta:
ordering = ['-updated']
get_latest_by = 'updated'
verbose_name = _('Topic')
verbose_name_plural = _('Topics')
permissions = (
('delayed_close', 'Can close topics after a delay'),
)
def __unicode__(self):
return self.name
def move_to(self, new_forum):
"""
Move a topic to a new forum.
"""
self.clear_last_forum_post()
old_forum = self.forum
self.forum = new_forum
self.save()
old_forum.set_last_post()
old_forum.set_counts()
old_forum.save()
def delete(self, *args, **kwargs):
self.clear_last_forum_post()
forum = self.forum
if forum_settings.SOFT_DELETE_TOPICS and (self.forum != get_object_or_404(Forum, pk=forum_settings.SOFT_DELETE_TOPICS) or not kwargs.get('staff', False)):
self.forum = get_object_or_404(Forum, pk=forum_settings.SOFT_DELETE_TOPICS)
self.save()
else:
super(Topic, self).delete()
forum.set_last_post()
forum.set_counts()
forum.save()
@property
def head(self):
try:
return self.posts.select_related().order_by('created')[0]
except IndexError:
return None
@property
def reply_count(self):
return self.post_count - 1
@models.permalink
def get_absolute_url(self):
return ('djangobb:topic', [self.id])
def get_mobile_url(self):
return reverse('djangobb:mobile_topic', args=[self.id])
def update_read(self, user):
tracking = user.posttracking
#if last_read > last_read - don't check topics
if tracking.last_read and (tracking.last_read > self.last_post.created):
return
if isinstance(tracking.topics, dict):
#clear topics if len > 5Kb and set last_read to current time
if len(tracking.topics) > 5120:
tracking.topics = None
tracking.last_read = timezone.now()
tracking.save()
#update topics if exist new post or does't exist in dict
elif self.last_post_id > tracking.topics.get(str(self.id), 0):
tracking.topics[str(self.id)] = self.last_post_id
tracking.save()
else:
#initialize topic tracking dict
tracking.topics = {self.id: self.last_post_id}
tracking.save()
def clear_last_forum_post(self):
"""
Prep for moving/deleting. Update the forum the topic belongs to.
"""
try:
last_post = self.posts.latest()
last_post.last_forum_post.clear()
except Post.DoesNotExist:
pass
else:
last_post.last_forum_post.clear()
class Post(models.Model):
topic = models.ForeignKey(Topic, related_name='posts', verbose_name=_('Topic'))
user = models.ForeignKey(User, related_name='posts', verbose_name=_('User'))
created = models.DateTimeField(_('Created'), auto_now_add=True)
updated = models.DateTimeField(_('Updated'), blank=True, null=True)
updated_by = models.ForeignKey(User, verbose_name=_('Updated by'), blank=True, null=True)
markup = models.CharField(_('Markup'), max_length=15, default=forum_settings.DEFAULT_MARKUP, choices=MARKUP_CHOICES)
body = models.TextField(_('Message'), validators=[MaxLengthValidator(forum_settings.POST_MAX_LENGTH)])
body_html = models.TextField(_('HTML version'))
user_ip = models.IPAddressField(_('User IP'), blank=True, null=True)
class Meta:
ordering = ['created']
get_latest_by = 'created'
verbose_name = _('Post')
verbose_name_plural = _('Posts')
permissions = (
('fast_post', 'Can add posts without a time limit'),
('med_post', 'Can add posts at medium speed'),
('post_external_links', 'Can post external links'),
('delayed_delete', 'Can delete posts after a delay'),
)
def save(self, *args, **kwargs):
self.body_html = convert_text_to_html(self.body, self.user.forum_profile)
if forum_settings.SMILES_SUPPORT and self.user.forum_profile.show_smilies:
self.body_html = smiles(self.body_html)
super(Post, self).save(*args, **kwargs)
def move_to(self, to_topic, delete_topic=True):
delete_topic = (self.topic.posts.count() == 1) and delete_topic
prev_topic = self.topic
self.topic = to_topic
self.save()
self.set_counts()
if delete_topic:
prev_topic.delete()
prev_topic.forum.set_last_post()
prev_topic.forum.set_counts()
prev_topic.forum.save()
def delete(self, *args, **kwargs):
self_id = self.id
head_post_id = self.topic.posts.order_by('created')[0].id
forum = self.topic.forum
topic = self.topic
profile = self.user.forum_profile
self.last_topic_post.clear()
self.last_forum_post.clear()
# If we actually delete the post, we lose any reports that my have come from it. Also, there is no recovery (but I don't care about that as much right now)
if self_id == head_post_id:
topic.delete(*args, **kwargs)
else:
if forum_settings.SOFT_DELETE_POSTS and (self.topic != get_object_or_404(Topic, pk=forum_settings.SOFT_DELETE_POSTS) or not kwargs.get('staff', False)):
self.topic = get_object_or_404(Topic, pk=forum_settings.SOFT_DELETE_POSTS)
self.save()
else:
super(Post, self).delete()
#if post was last in topic - remove topic
try:
topic.last_post = Post.objects.filter(topic__id=topic.id).latest()
except Post.DoesNotExist:
topic.last_post = None
topic.post_count = Post.objects.filter(topic__id=topic.id).count()
topic.save()
forum.set_last_post()
forum.save()
self.set_counts()
def set_counts(self):
"""
Recounts this post's forum and and topic post counts.
"""
forum = self.topic.forum
profile = self.user.forum_profile
#TODO: for speedup - save/update only changed fields
forum.set_counts()
forum.save()
profile.set_counts()
profile.save()
@models.permalink
def get_absolute_url(self):
return ('djangobb:post', [self.id])
def get_mobile_url(self):
return reverse('djangobb:mobile_post', args=[self.id])
def summary(self):
LIMIT = 50
tail = len(self.body) > LIMIT and '...' or ''
return self.body[:LIMIT] + tail
__unicode__ = summary
class Reputation(models.Model):
from_user = models.ForeignKey(User, related_name='reputations_from', verbose_name=_('From'))
to_user = models.ForeignKey(User, related_name='reputations_to', verbose_name=_('To'))
post = models.ForeignKey(Post, related_name='post', verbose_name=_('Post'))
time = models.DateTimeField(_('Time'), auto_now_add=True)
sign = models.IntegerField(_('Sign'), choices=SIGN_CHOICES, default=0)
reason = models.TextField(_('Reason'), max_length=1000)
class Meta:
verbose_name = _('Reputation')
verbose_name_plural = _('Reputations')
unique_together = (('from_user', 'post'),)
def __unicode__(self):
return u'T[%d], FU[%d], TU[%d]: %s' % (self.post.id, self.from_user.id, self.to_user.id, unicode(self.time))
class ProfileManager(models.Manager):
use_for_related_fields = True
def get_query_set(self):
qs = super(ProfileManager, self).get_query_set()
if forum_settings.REPUTATION_SUPPORT:
qs = qs.extra(select={
'reply_total': 'SELECT SUM(sign) FROM djangobb_forum_reputation WHERE to_user_id = djangobb_forum_profile.user_id GROUP BY to_user_id',
'reply_count_minus': "SELECT SUM(sign) FROM djangobb_forum_reputation WHERE to_user_id = djangobb_forum_profile.user_id AND sign = '-1' GROUP BY to_user_id",
'reply_count_plus': "SELECT SUM(sign) FROM djangobb_forum_reputation WHERE to_user_id = djangobb_forum_profile.user_id AND sign = '1' GROUP BY to_user_id",
})
return qs
class Profile(models.Model):
user = AutoOneToOneField(User, related_name='forum_profile', verbose_name=_('User'))
status = models.CharField(_('Status'), max_length=30, blank=True)
site = models.URLField(_('Site'), verify_exists=False, blank=True)
jabber = models.CharField(_('Jabber'), max_length=80, blank=True)
icq = models.CharField(_('ICQ'), max_length=12, blank=True)
msn = models.CharField(_('MSN'), max_length=80, blank=True)
aim = models.CharField(_('AIM'), max_length=80, blank=True)
yahoo = models.CharField(_('Yahoo'), max_length=80, blank=True)
location = models.CharField(_('Location'), max_length=30, blank=True)
signature = models.TextField(_('Signature'), blank=True, default='', max_length=forum_settings.SIGNATURE_MAX_LENGTH)
signature_html = models.TextField(_('Signature'), blank=True, default='', max_length=forum_settings.SIGNATURE_MAX_LENGTH)
time_zone = models.FloatField(_('Time zone'), choices=TZ_CHOICES, default=float(forum_settings.DEFAULT_TIME_ZONE))
language = models.CharField(_('Language'), max_length=5, default='', choices=settings.LANGUAGES)
avatar = ExtendedImageField(_('Avatar'), blank=True, default='', upload_to=forum_settings.AVATARS_UPLOAD_TO, width=forum_settings.AVATAR_WIDTH, height=forum_settings.AVATAR_HEIGHT)
theme = models.CharField(_('Theme'), choices=THEME_CHOICES, max_length=80, default='default')
show_avatar = models.BooleanField(_('Show avatar'), blank=True, default=True)
show_signatures = models.BooleanField(_('Show signatures'), blank=True, default=True)
show_smilies = models.BooleanField(_('Show smilies'), blank=True, default=True)
privacy_permission = models.IntegerField(_('Privacy permission'), choices=PRIVACY_CHOICES, default=1)
auto_subscribe = models.BooleanField(_('Auto subscribe'), help_text=_("Auto subscribe all topics you have created or reply."), blank=True, default=False)
markup = models.CharField(_('Default markup'), max_length=15, default=forum_settings.DEFAULT_MARKUP, choices=MARKUP_CHOICES)
post_count = models.IntegerField(_('Post count'), blank=True, default=0)
objects = ProfileManager()
class Meta:
verbose_name = _('Profile')
verbose_name_plural = _('Profiles')
def last_post(self):
posts = Post.objects.filter(user__id=self.user_id).order_by('-created')
if posts:
return posts[0].created
else:
return None
def set_counts(self):
self.post_count = Post.objects.filter(user=self.user).count()
class PostTracking(models.Model):
"""
Model for tracking read/unread posts.
In topics stored ids of topics and last_posts as dict.
"""
user = AutoOneToOneField(User)
topics = JSONField(null=True, blank=True)
last_read = models.DateTimeField(null=True, blank=True)
class Meta:
verbose_name = _('Post tracking')
verbose_name_plural = _('Post tracking')
def __unicode__(self):
return self.user.username
class Report(models.Model):
reported_by = models.ForeignKey(User, related_name='reported_by', verbose_name=_('Reported by'))
post = models.ForeignKey(Post, verbose_name=_('Post'))
zapped = models.BooleanField(_('Zapped'), blank=True, default=False)
zapped_by = models.ForeignKey(User, related_name='zapped_by', blank=True, null=True, verbose_name=_('Zapped by'))
created = models.DateTimeField(_('Created'), blank=True)
reason = models.TextField(_('Reason'), blank=True, default='', max_length='1000')
class Meta:
verbose_name = _('Report')
verbose_name_plural = _('Reports')
def __unicode__(self):
return u'%s %s' % (self.reported_by , self.zapped)
class Ban(models.Model):
user = models.OneToOneField(User, verbose_name=_('Banned user'), related_name='ban_users')
ban_start = models.DateTimeField(_('Ban start'), default=timezone.now)
ban_end = models.DateTimeField(_('Ban end'), blank=True, null=True)
reason = models.TextField(_('Reason'))
class Meta:
verbose_name = _('Ban')
verbose_name_plural = _('Bans')
def __unicode__(self):
return self.user.username
def save(self, *args, **kwargs):
self.user.is_active = False
self.user.save()
super(Ban, self).save(*args, **kwargs)
def delete(self, *args, **kwargs):
self.user.is_active = True
self.user.save()
super(Ban, self).delete(*args, **kwargs)
class Attachment(models.Model):
post = models.ForeignKey(Post, verbose_name=_('Post'), related_name='attachments')
size = models.IntegerField(_('Size'))
content_type = models.CharField(_('Content type'), max_length=255)
path = models.CharField(_('Path'), max_length=255)
name = models.TextField(_('Name'))
hash = models.CharField(_('Hash'), max_length=40, blank=True, default='', db_index=True)
def __unicode__(self):
return self.name
def save(self, *args, **kwargs):
super(Attachment, self).save(*args, **kwargs)
if not self.hash:
self.hash = sha1(str(self.id) + settings.SECRET_KEY).hexdigest()
super(Attachment, self).save(*args, **kwargs)
@models.permalink
def get_absolute_url(self):
return ('djangobb:forum_attachment', [self.hash])
def get_absolute_path(self):
return os.path.join(settings.MEDIA_ROOT, forum_settings.ATTACHMENT_UPLOAD_TO,
self.path)
#------------------------------------------------------------------------------
class Poll(models.Model):
topic = models.ForeignKey(Topic)
question = models.CharField(max_length=200)
choice_count = models.PositiveSmallIntegerField(default=1,
help_text=_("How many choices are allowed simultaneously."),
)
active = models.BooleanField(default=True,
help_text=_("Can users vote to this poll or just see the result?"),
)
deactivate_date = models.DateTimeField(null=True, blank=True,
help_text=_("Point of time after this poll would be automatic deactivated"),
)
users = models.ManyToManyField(User, blank=True, null=True,
help_text=_("Users who has voted this poll."),
)
def auto_deactivate(self):
if self.active and self.deactivate_date:
now = timezone.now()
if now > self.deactivate_date:
self.active = False
self.save()
def __unicode__(self):
return self.question
class PollChoice(models.Model):
poll = models.ForeignKey(Poll, related_name="choices")
choice = models.CharField(max_length=200)
votes = models.IntegerField(default=0, editable=False)
def percent(self):
if not self.votes:
return 0.0
result = PollChoice.objects.filter(poll=self.poll).aggregate(aggregates.Sum("votes"))
votes_sum = result["votes__sum"]
return float(self.votes) / votes_sum * 100
def __unicode__(self):
return self.choice
#------------------------------------------------------------------------------
class PostStatusManager(models.Manager):
def create_for_post(self, post, **kwargs):
user_agent = kwargs.get("HTTP_USER_AGENT", None)
referrer = kwargs.get("HTTP_REFERER", None)
permalink = kwargs.get("permalink", None)
return self.create(
post=post, topic=post.topic, forum=post.topic.forum,
user_agent=user_agent, referrer=referrer, permalink=permalink)
def review_posts(self, posts, certainly_spam=False):
for post in posts:
try:
post_status = post.poststatus
except PostStatus.DoesNotExist:
post_status = self.create_for_post(post)
post_status.review(certainly_spam=certainly_spam)
def delete_user_posts(self, posts):
for post in posts:
try:
post_status = post.poststatus
except PostStatus.DoesNotExist:
post_status = self.create_for_post(post)
post_status.filter_user_deleted()
def undelete_user_posts(self, posts):
for post in posts:
try:
post_status = post.poststatus
except PostStatus.DoesNotExist:
post_status = self.create_for_post(post)
post_status.filter_user_undeleted()
def review_new_posts(self):
unreviewed = self.filter(state=PostStatus.UNREVIEWED)
for post_status in unreviewed:
post_status.review()
return unreviewed
class PostStatus(models.Model):
"""
Keeps track of the status of posts for moderation purposes.
"""
UNREVIEWED = 'unreviewed'
USER_DELETED = 'user_deleted'
FILTERED_SPAM = 'filtered_spam'
FILTERED_HAM = 'filtered_ham'
MARKED_SPAM = 'marked_spam'
MARKED_HAM = 'marked_ham'
AKISMET_MAX_SIZE = 1024*250
post = models.OneToOneField(Post, db_index=True)
state = FSMField(default=UNREVIEWED, db_index=True)
topic = models.ForeignKey(Topic) # Original topic
forum = models.ForeignKey(Forum) # Original forum
user_agent = models.CharField(max_length=200, blank=True, null=True)
referrer = models.CharField(max_length=200, blank=True, null=True)
permalink = models.CharField(max_length=200, blank=True, null=True)
objects = PostStatusManager()
spam_category = None
spam_forum = None
spam_topic = None
def _get_spam_dustbin(self):
if self.spam_category is None:
self.spam_category, _ = Category.objects.get_or_create(
name=forum_settings.SPAM_CATEGORY_NAME)
if self.spam_forum is None:
self.spam_forum, _ = Forum.objects.get_or_create(
category=self.spam_category,
name=forum_settings.SPAM_FORUM_NAME)
if self.spam_topic is None:
filterbot = User.objects.get_by_natural_key("filterbot")
self.spam_topic, _ = Topic.objects.get_or_create(
forum=self.spam_forum, name=forum_settings.SPAM_TOPIC_NAME,
user=filterbot)
return (self.spam_topic, self.spam_forum)
def _undelete_post(self):
"""
If the post is in the spam dustbin, move it back to its original location.
"""
spam_topic, spam_forum = self._get_spam_dustbin()
post = self.post
topic = self.topic
head = post.topic.head
if post == head:
# Move the original topic back to the original forum (either from
# the dustbin, or from the spam dustbin)
topic.move_to(self.forum)
if topic != post.topic:
# If the post was moved from its original topic, put it back now that
# the topic is in place.
post.move_to(topic, delete_topic=False)
def _delete_post(self):
"""
Move the post to the spam dustbin.
"""
spam_topic, spam_forum = self._get_spam_dustbin()
post = self.post
topic = self.topic
head = topic.head
if post == head:
topic.move_to(spam_forum)
else:
post.move_to(spam_topic)
def to_akismet_data(self):
post = self.post
topic = self.topic
user = post.user
user_ip = post.user_ip
comment_author = user.username
user_agent = self.user_agent
referrer = self.referrer
permalink = self.permalink
comment_date_gmt = post.created.isoformat(' ')
comment_post_modified_gmt = topic.created.isoformat(' ')
return {
'user_ip': user_ip,
'user_agent': user_agent,
'comment_author': comment_author,
'referrer': referrer,
'permalink': permalink,
'comment_type': 'comment',
'comment_date_gmt': comment_date_gmt,
'comment_post_modified_gmt': comment_post_modified_gmt
}
def to_akismet_content(self):
"""
Truncate the post body to the largest allowed string size. Use size, not
length, since the Akismet server checks size, not length.
"""
return self.post.body.encode('utf-8')[:self.AKISMET_MAX_SIZE].decode('utf-8', 'ignore')
def _comment_check(self):
"""
Pass the associated post through Akismet if it's available. If it's not
available return None. Otherwise return True or False.
"""
if akismet_api is None:
logger.warning("Skipping akismet check. No api.")
return None
data = self.to_akismet_data()
content = self.to_akismet_content()
is_spam = None
try:
is_spam = akismet_api.comment_check(content, data)
except AkismetError as e:
try:
# try again, in case of timeout
is_spam = akismet_api.comment_check(content, data)
except Exception as e:
logger.error(
"Error while checking Akismet", exc_info=True, extra={
"post": self.post, "post_id": self.post.id,
"content_length": len(content)})
is_spam = None
except Exception as e:
logger.error(
"Error while checking Akismet", exc_info=True, extra={
"post": self.post, "post_id": self.post.id,
"content_length": len(content)})
is_spam = None
return is_spam
def _submit_comment(self, report_type):
"""
Report this post to Akismet as spam or ham. Raises an exception if it
fails. report_type is 'spam' or 'ham'. Used by report_spam/report_ham.
"""
if akismet_api is None:
logger.error("Can't submit to Akismet. No API.")
return None
data = self.to_akismet_data()
content = self.to_akismet_content()
if report_type == "spam":
akismet_api.submit_spam(content, data)
elif report_type == "ham":
akismet_api.submit_ham(content, data)
else:
raise NotImplementedError(
"You're trying to report an unsupported comment type.")
def _submit_spam(self):
"""
Report this post to Akismet as spam.
"""
self._submit_comment("spam")
def _submit_ham(self):
"""
Report this post to Akismet as ham.
"""
self._submit_comment("ham")
def is_spam(self):
"""
Condition used by the FSM. Return True if the Akismet API is available
and returns a positive. Otherwise return False or None.
"""
is_spam = self._comment_check()
if is_spam is None:
return False
else:
return is_spam
def is_ham(self):
"""
Inverse of is_spam.
"""
is_spam = self._comment_check()
if is_spam is None:
return False
else:
return not is_spam
@transition(
field=state, source=UNREVIEWED, target=FILTERED_SPAM,
save=True, conditions=[is_spam])
def filter_spam(self):
"""
Akismet detected this post is spam, move it to the dustbin and report it.
"""
self._delete_post()
@transition(
field=state, source=UNREVIEWED, target=FILTERED_HAM,
save=True, conditions=[is_ham])
def filter_ham(self):
"""
Akismet detected this post as ham. Don't do anything (except change state).
"""
pass
@transition(
field=state, source=[UNREVIEWED, FILTERED_HAM, MARKED_HAM], target=USER_DELETED,
save=True)
def filter_user_deleted(self):
"""
Post is not marked spam by akismet, but user has been globally deleted,
putting this into the spam dusbin.
"""
self._delete_post()
@transition(
field=state, source=[FILTERED_SPAM, MARKED_SPAM], target=MARKED_HAM,
save=True)
def mark_ham(self):
"""
Either Akismet returned a false positive, or a moderator accidentally
marked this as spam. Tell Akismet that this is ham, undelete it.
"""
self._submit_ham()
self._undelete_post()
@transition(
field=state, source=[FILTERED_HAM, MARKED_HAM], target=MARKED_SPAM,
save=True)
def mark_spam(self):
"""
Akismet missed this, or a moderator accidentally marked it as ham. Tell
Akismet that this is spam.
"""
self._submit_spam()
self._delete_post()
@transition(
field=state, source=USER_DELETED, target=UNREVIEWED,
save=True)
def filter_user_undeleted(self):
"""
Post is not marked spam by akismet, but user has been globally deleted,
putting this into the spam dusbin.
"""
self._undelete_post()
def review(self, certainly_spam=False):
"""
Process this post, used by the manager and the spam-hammer. The
``certainly_spam`` argument is used to force mark as spam/delete the
post, no matter what status Akismet returns.
"""
if can_proceed(self.filter_spam):
self.filter_spam()
elif can_proceed(self.filter_ham):
self.filter_ham()
if certainly_spam:
self.mark_spam()
else:
if certainly_spam:
self._delete_post()
logger.warn(
"Couldn't filter post.", exc_info=True, extra={
'post_id': self.post.id, 'content_length': len(self.post.body)})
from .signals import post_saved, topic_saved
post_save.connect(post_saved, sender=Post, dispatch_uid='djangobb_post_save')
post_save.connect(topic_saved, sender=Topic, dispatch_uid='djangobb_topic_save')
| tjvr/s2forums | djangobb_forum/models.py | Python | bsd-3-clause | 32,814 |
"""
The main site views
"""
__author__='Martin Knobel'
| chipperdoodles/3color | threecolor/site/__init__.py | Python | bsd-3-clause | 57 |
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2017-11-10 17:32
from __future__ import unicode_literals
from django.db import migrations, models
import django.db.models.deletion
import filebrowser.fields
class Migration(migrations.Migration):
dependencies = [
('subscriptions', '0002_auto_20171009_1949'),
]
operations = [
migrations.AlterModelOptions(
name='subscription',
options={'verbose_name': 'Subscription', 'verbose_name_plural': 'Subscriptions'},
),
migrations.AlterModelOptions(
name='subscriptionevent',
options={'verbose_name': 'Subscription Event', 'verbose_name_plural': 'Subscription Events'},
),
migrations.AlterModelOptions(
name='subscriptiontype',
options={'verbose_name': 'Subscription Type', 'verbose_name_plural': 'Subscription Types'},
),
migrations.AlterField(
model_name='subscription',
name='contract',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='crm.Contract', verbose_name='Subscription Type'),
),
migrations.AlterField(
model_name='subscription',
name='subscriptiontype',
field=models.ForeignKey(null=True, on_delete=django.db.models.deletion.CASCADE, to='subscriptions.SubscriptionType', verbose_name='Subscription Type'),
),
migrations.AlterField(
model_name='subscriptionevent',
name='event',
field=models.CharField(choices=[('O', 'Offered'), ('C', 'Canceled'), ('S', 'Signed')], max_length=1, verbose_name='Event'),
),
migrations.AlterField(
model_name='subscriptionevent',
name='eventdate',
field=models.DateField(blank=True, null=True, verbose_name='Event Date'),
),
migrations.AlterField(
model_name='subscriptionevent',
name='subscriptions',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='subscriptions.Subscription', verbose_name='Subscription'),
),
migrations.AlterField(
model_name='subscriptiontype',
name='automaticContractExtension',
field=models.IntegerField(blank=True, null=True, verbose_name='Automatic Contract Extension (months)'),
),
migrations.AlterField(
model_name='subscriptiontype',
name='automaticContractExtensionReminder',
field=models.IntegerField(blank=True, null=True, verbose_name='Automatic Contract Extensoin Reminder (days)'),
),
migrations.AlterField(
model_name='subscriptiontype',
name='cancelationPeriod',
field=models.IntegerField(blank=True, null=True, verbose_name='Cancelation Period (months)'),
),
migrations.AlterField(
model_name='subscriptiontype',
name='contractDocument',
field=filebrowser.fields.FileBrowseField(blank=True, max_length=200, null=True, verbose_name='Contract Documents'),
),
migrations.AlterField(
model_name='subscriptiontype',
name='minimumDuration',
field=models.IntegerField(blank=True, null=True, verbose_name='Minimum Contract Duration'),
),
migrations.AlterField(
model_name='subscriptiontype',
name='paymentIntervall',
field=models.IntegerField(blank=True, null=True, verbose_name='Payment Intervall (days)'),
),
]
| scaphilo/koalixcrm | koalixcrm/subscriptions/migrations/0003_auto_20171110_1732.py | Python | bsd-3-clause | 3,603 |
# -*- coding: utf-8 -*-
from __future__ import with_statement
from cms.management.commands import publisher_publish
from cms.models.pagemodel import Page
from cms.test.testcases import CMSTestCase
from cms.test.util.context_managers import SettingsOverride, StdoutOverride
from django.contrib.auth.models import User
from django.core.management.base import CommandError
class PublisherTestCase(CMSTestCase):
'''
A test case to exercise publisher
'''
def test_01_simple_publisher(self):
'''
Creates the stuff needed for theses tests.
Please keep this up-to-date (the docstring!)
A
/ \
B C
'''
# Create a simple tree of 3 pages
pageA = self.create_page(title="Page A", published= True,
in_navigation= True)
pageB = self.create_page(parent_page=pageA,title="Page B",
published= True, in_navigation= True)
pageC = self.create_page(parent_page=pageA,title="Page C",
published= False, in_navigation= True)
# Assert A and B are published, C unpublished
self.assertTrue(pageA.published)
self.assertTrue(pageB.published)
self.assertTrue(not pageC.published)
self.assertTrue(len(Page.objects.published()), 2)
# Let's publish C now.
pageC.publish()
# Assert A and B are published, C unpublished
self.assertTrue(pageA.published)
self.assertTrue(pageB.published)
self.assertTrue(pageC.published)
self.assertTrue(len(Page.objects.published()), 3)
def test_02_command_line_should_raise_without_superuser(self):
raised = False
try:
com = publisher_publish.Command()
com.handle_noargs()
except CommandError:
raised = True
self.assertTrue(raised)
def test_03_command_line_should_raise_when_moderator_false(self):
with SettingsOverride(CMS_MODERATOR=False):
raised = False
try:
com = publisher_publish.Command()
com.handle_noargs()
except CommandError:
raised = True
self.assertTrue(raised)
def test_04_command_line_publishes_zero_pages_on_empty_db(self):
# we need to create a superuser (the db is empty)
User.objects.create_superuser('djangocms', '[email protected]', '123456')
pages_from_output = 0
published_from_output = 0
with StdoutOverride() as buffer:
# Now we don't expect it to raise, but we need to redirect IO
com = publisher_publish.Command()
com.handle_noargs()
lines = buffer.getvalue().split('\n') #NB: readlines() doesn't work
for line in lines:
if 'Total' in line:
pages_from_output = int(line.split(':')[1])
elif 'Published' in line:
published_from_output = int(line.split(':')[1])
self.assertEqual(pages_from_output,0)
self.assertEqual(published_from_output,0)
def test_05_command_line_publishes_one_page(self):
'''
Publisher always creates two Page objects for every CMS page,
one is_draft and one is_public.
The public version of the page can be either published or not.
This bit of code uses sometimes manager methods and sometimes manual
filters on purpose (this helps test the managers)
'''
# we need to create a superuser (the db is empty)
User.objects.create_superuser('djangocms', '[email protected]', '123456')
# Now, let's create a page. That actually creates 2 Page objects
self.create_page(title="The page!", published=True,
in_navigation=True)
draft = Page.objects.drafts()[0]
draft.reverse_id = 'a_test' # we have to change *something*
draft.save()
pages_from_output = 0
published_from_output = 0
with StdoutOverride() as buffer:
# Now we don't expect it to raise, but we need to redirect IO
com = publisher_publish.Command()
com.handle_noargs()
lines = buffer.getvalue().split('\n') #NB: readlines() doesn't work
for line in lines:
if 'Total' in line:
pages_from_output = int(line.split(':')[1])
elif 'Published' in line:
published_from_output = int(line.split(':')[1])
self.assertEqual(pages_from_output,1)
self.assertEqual(published_from_output,1)
# Sanity check the database (we should have one draft and one public)
not_drafts = len(Page.objects.filter(publisher_is_draft=False))
drafts = len(Page.objects.filter(publisher_is_draft=True))
self.assertEquals(not_drafts,1)
self.assertEquals(drafts,1)
# Now check that the non-draft has the attribute we set to the draft.
non_draft = Page.objects.public()[0]
self.assertEquals(non_draft.reverse_id, 'a_test')
def test_06_unpublish(self):
page = self.create_page(title="Page", published=True,
in_navigation=True)
page.published = False
page.save()
self.assertEqual(page.published, False)
page.published = True
page.save()
self.assertEqual(page.published, True)
def test_07_publish_works_with_descendants(self):
'''
For help understanding what this tests for, see:
http://articles.sitepoint.com/print/hierarchical-data-database
'''
home_page = self.create_page(title="home", published=True,
in_navigation=False)
item1 = self.create_page(title="item1", parent_page=home_page,
published=True)
item2 = self.create_page(title="item2", parent_page=home_page,
published=True)
item2 = self.reload_page(item2)
subitem1 = self.create_page(title="subitem1", parent_page=item2,
published=True)
subitem2 = self.create_page(title="subitem2", parent_page=item2,
published=True)
not_drafts = list(Page.objects.filter(publisher_is_draft=False).order_by('lft'))
drafts = list(Page.objects.filter(publisher_is_draft=True).order_by('lft'))
self.assertEquals(len(not_drafts), 5)
self.assertEquals(len(drafts), 5)
for idx, draft in enumerate(drafts):
public = not_drafts[idx]
# Check that a node doesn't become a root node magically
self.assertEqual(bool(public.parent_id), bool(draft.parent_id))
if public.parent :
# Let's assert the MPTT tree is consistent
self.assertTrue(public.lft > public.parent.lft)
self.assertTrue(public.rght < public.parent.rght)
self.assertEquals(public.tree_id, public.parent.tree_id)
self.assertTrue(public.parent in public.get_ancestors())
self.assertTrue(public in public.parent.get_descendants())
self.assertTrue(public in public.parent.get_children())
if draft.parent:
# Same principle for the draft tree
self.assertTrue(draft.lft > draft.parent.lft)
self.assertTrue(draft.rght < draft.parent.rght)
self.assertEquals(draft.tree_id, draft.parent.tree_id)
self.assertTrue(draft.parent in draft.get_ancestors())
self.assertTrue(draft in draft.parent.get_descendants())
self.assertTrue(draft in draft.parent.get_children())
item2 = self.reload_page(item2)
item2.publish()
not_drafts = list(Page.objects.filter(publisher_is_draft=False).order_by('lft'))
drafts = list(Page.objects.filter(publisher_is_draft=True).order_by('lft'))
self.assertEquals(len(not_drafts), 5)
self.assertEquals(len(drafts), 5)
for idx, draft in enumerate(drafts):
public = not_drafts[idx]
# Check that a node doesn't become a root node magically
self.assertEqual(bool(public.parent_id), bool(draft.parent_id))
if public.parent :
# Let's assert the MPTT tree is consistent
self.assertTrue(public.lft > public.parent.lft)
self.assertTrue(public.rght < public.parent.rght)
self.assertEquals(public.tree_id, public.parent.tree_id)
self.assertTrue(public.parent in public.get_ancestors())
self.assertTrue(public in public.parent.get_descendants())
self.assertTrue(public in public.parent.get_children())
if draft.parent:
# Same principle for the draft tree
self.assertTrue(draft.lft > draft.parent.lft)
self.assertTrue(draft.rght < draft.parent.rght)
self.assertEquals(draft.tree_id, draft.parent.tree_id)
self.assertTrue(draft.parent in draft.get_ancestors())
self.assertTrue(draft in draft.parent.get_descendants())
self.assertTrue(draft in draft.parent.get_children())
| team-xue/xue | xue/cms/tests/publisher.py | Python | bsd-3-clause | 9,591 |
# -*- coding: utf-8 -*-
#
# Copyright (c) 2015, Alcatel-Lucent Inc, 2017 Nokia
# All rights reserved.
#
# Redistribution and use in source and binary forms, with or without
# modification, are permitted provided that the following conditions are met:
# * Redistributions of source code must retain the above copyright
# notice, this list of conditions and the following disclaimer.
# * Redistributions in binary form must reproduce the above copyright
# notice, this list of conditions and the following disclaimer in the
# documentation and/or other materials provided with the distribution.
# * Neither the name of the copyright holder nor the names of its contributors
# may be used to endorse or promote products derived from this software without
# specific prior written permission.
#
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
# ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
# WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
# DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY
# DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
# (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
# LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
# ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
# (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
# SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
from bambou import NURESTFetcher
class NUQosPolicersFetcher(NURESTFetcher):
""" Represents a NUQosPolicers fetcher
Notes:
This fetcher enables to fetch NUQosPolicer objects.
See:
bambou.NURESTFetcher
"""
@classmethod
def managed_class(cls):
""" Return NUQosPolicer class that is managed.
Returns:
.NUQosPolicer: the managed class
"""
from .. import NUQosPolicer
return NUQosPolicer
| nuagenetworks/vspk-python | vspk/v6/fetchers/nuqospolicers_fetcher.py | Python | bsd-3-clause | 2,121 |
#!/usr/bin/env python
__version__ = '0.8.9'
| eufarn7sp/egads-eufar | egads/_version.py | Python | bsd-3-clause | 44 |
import numpy as np
import pandas as pd
import pandas._testing as tm
from .base import BaseExtensionTests
class BaseMissingTests(BaseExtensionTests):
def test_isna(self, data_missing):
expected = np.array([True, False])
result = pd.isna(data_missing)
tm.assert_numpy_array_equal(result, expected)
result = pd.Series(data_missing).isna()
expected = pd.Series(expected)
self.assert_series_equal(result, expected)
# GH 21189
result = pd.Series(data_missing).drop([0, 1]).isna()
expected = pd.Series([], dtype=bool)
self.assert_series_equal(result, expected)
def test_dropna_array(self, data_missing):
result = data_missing.dropna()
expected = data_missing[[1]]
self.assert_extension_array_equal(result, expected)
def test_dropna_series(self, data_missing):
ser = pd.Series(data_missing)
result = ser.dropna()
expected = ser.iloc[[1]]
self.assert_series_equal(result, expected)
def test_dropna_frame(self, data_missing):
df = pd.DataFrame({"A": data_missing})
# defaults
result = df.dropna()
expected = df.iloc[[1]]
self.assert_frame_equal(result, expected)
# axis = 1
result = df.dropna(axis="columns")
expected = pd.DataFrame(index=[0, 1])
self.assert_frame_equal(result, expected)
# multiple
df = pd.DataFrame({"A": data_missing, "B": [1, np.nan]})
result = df.dropna()
expected = df.iloc[:0]
self.assert_frame_equal(result, expected)
def test_fillna_scalar(self, data_missing):
valid = data_missing[1]
result = data_missing.fillna(valid)
expected = data_missing.fillna(valid)
self.assert_extension_array_equal(result, expected)
def test_fillna_limit_pad(self, data_missing):
arr = data_missing.take([1, 0, 0, 0, 1])
result = pd.Series(arr).fillna(method="ffill", limit=2)
expected = pd.Series(data_missing.take([1, 1, 1, 0, 1]))
self.assert_series_equal(result, expected)
def test_fillna_limit_backfill(self, data_missing):
arr = data_missing.take([1, 0, 0, 0, 1])
result = pd.Series(arr).fillna(method="backfill", limit=2)
expected = pd.Series(data_missing.take([1, 0, 1, 1, 1]))
self.assert_series_equal(result, expected)
def test_fillna_series(self, data_missing):
fill_value = data_missing[1]
ser = pd.Series(data_missing)
result = ser.fillna(fill_value)
expected = pd.Series(
data_missing._from_sequence(
[fill_value, fill_value], dtype=data_missing.dtype
)
)
self.assert_series_equal(result, expected)
# Fill with a series
result = ser.fillna(expected)
self.assert_series_equal(result, expected)
# Fill with a series not affecting the missing values
result = ser.fillna(ser)
self.assert_series_equal(result, ser)
def test_fillna_series_method(self, data_missing, fillna_method):
fill_value = data_missing[1]
if fillna_method == "ffill":
data_missing = data_missing[::-1]
result = pd.Series(data_missing).fillna(method=fillna_method)
expected = pd.Series(
data_missing._from_sequence(
[fill_value, fill_value], dtype=data_missing.dtype
)
)
self.assert_series_equal(result, expected)
def test_fillna_frame(self, data_missing):
fill_value = data_missing[1]
result = pd.DataFrame({"A": data_missing, "B": [1, 2]}).fillna(fill_value)
expected = pd.DataFrame(
{
"A": data_missing._from_sequence(
[fill_value, fill_value], dtype=data_missing.dtype
),
"B": [1, 2],
}
)
self.assert_frame_equal(result, expected)
def test_fillna_fill_other(self, data):
result = pd.DataFrame({"A": data, "B": [np.nan] * len(data)}).fillna({"B": 0.0})
expected = pd.DataFrame({"A": data, "B": [0.0] * len(result)})
self.assert_frame_equal(result, expected)
def test_use_inf_as_na_no_effect(self, data_missing):
ser = pd.Series(data_missing)
expected = ser.isna()
with pd.option_context("mode.use_inf_as_na", True):
result = ser.isna()
self.assert_series_equal(result, expected)
| jreback/pandas | pandas/tests/extension/base/missing.py | Python | bsd-3-clause | 4,515 |
import flux
import forge
from flux.gevent_timeline import GeventTimeline
from .test__timeline import TimeFactorTest, CurrentTimeLineTest, DatetimeTest, ScheduleSequenceTest, ScheduleTest, TimelineAPITest
class GeventTimeFactorTest(TimeFactorTest):
def _forge_timeline(self):
self.forge = forge.Forge()
self.forge.replace_with(GeventTimeline, "_real_time", self.time)
self.forge.replace_with(GeventTimeline, "_real_sleep", self.sleep)
self.timeline = GeventTimeline()
class GeventCurrentTimeLineTest(CurrentTimeLineTest):
def setUp(self):
super(GeventCurrentTimeLineTest, self).setUp()
timeline = GeventTimeline()
self.addCleanup(flux.current_timeline.set, flux.current_timeline.get())
flux.current_timeline.set(timeline)
class GeventScheduleSequenceTest(ScheduleSequenceTest):
def _get_timeline(self):
return GeventTimeline()
class GeventDatetimeTest(DatetimeTest):
def _get_timeline(self):
timeline = GeventTimeline()
self.addCleanup(flux.current_timeline.set, flux.current_timeline.get())
flux.current_timeline.set(timeline)
return timeline
class GeventScheduleTest(ScheduleTest):
def _get_timeline(self):
return GeventTimeline()
class GeventTimelineAPITest(TimelineAPITest):
def _get_timeline(self):
return GeventTimeline()
| omergertel/flux | tests/test__gevent_timeline.py | Python | bsd-3-clause | 1,377 |
# Copyright (c) 2008-2015 MetPy Developers.
# Distributed under the terms of the BSD 3-Clause License.
# SPDX-License-Identifier: BSD-3-Clause
"""Contains parsed specs for various NEXRAD messages formats."""
| ahill818/MetPy | metpy/io/_nexrad_msgs/__init__.py | Python | bsd-3-clause | 208 |
import mesh.patch as patch
import mesh.array_indexer as ai
import numpy as np
from numpy.testing import assert_array_equal
# utilities
def test_buf_split():
assert_array_equal(ai._buf_split(2), [2, 2, 2, 2])
assert_array_equal(ai._buf_split((2, 3)), [2, 3, 2, 3])
# ArrayIndexer tests
def test_indexer():
g = patch.Grid2d(2, 3, ng=2)
a = g.scratch_array()
a[:, :] = np.arange(g.qx*g.qy).reshape(g.qx, g.qy)
assert_array_equal(a.v(), np.array([[16., 17., 18.], [23., 24., 25.]]))
assert_array_equal(a.ip(1), np.array([[23., 24., 25.], [30., 31., 32.]]))
assert_array_equal(a.ip(-1), np.array([[9., 10., 11.], [16., 17., 18.]]))
assert_array_equal(a.jp(1), np.array([[17., 18., 19.], [24., 25., 26.]]))
assert_array_equal(a.jp(-1), np.array([[15., 16., 17.], [22., 23., 24.]]))
assert_array_equal(a.ip_jp(1, 1), np.array([[24., 25., 26.], [31., 32., 33.]]))
def test_is_symmetric():
g = patch.Grid2d(4, 3, ng=0)
a = g.scratch_array()
a[:, 0] = [1, 2, 2, 1]
a[:, 1] = [2, 4, 4, 2]
a[:, 2] = [1, 2, 2, 1]
assert a.is_symmetric()
def test_is_asymmetric():
g = patch.Grid2d(4, 3, ng=0)
a = g.scratch_array()
a[:, 0] = [-1, -2, 2, 1]
a[:, 1] = [-2, -4, 4, 2]
a[:, 2] = [-1, -2, 2, 1]
assert a.is_asymmetric()
| zingale/pyro2 | mesh/tests/test_array_indexer.py | Python | bsd-3-clause | 1,312 |
import logging
import posixpath
import warnings
from collections import defaultdict
from django.utils.deprecation import RemovedInDjango21Warning
from django.utils.safestring import mark_safe
from .base import (
Node, Template, TemplateSyntaxError, TextNode, Variable, token_kwargs,
)
from .library import Library
register = Library()
BLOCK_CONTEXT_KEY = 'block_context'
logger = logging.getLogger('django.template')
class BlockContext:
def __init__(self):
# Dictionary of FIFO queues.
self.blocks = defaultdict(list)
def add_blocks(self, blocks):
for name, block in blocks.items():
self.blocks[name].insert(0, block)
def pop(self, name):
try:
return self.blocks[name].pop()
except IndexError:
return None
def push(self, name, block):
self.blocks[name].append(block)
def get_block(self, name):
try:
return self.blocks[name][-1]
except IndexError:
return None
class BlockNode(Node):
def __init__(self, name, nodelist, parent=None):
self.name, self.nodelist, self.parent = name, nodelist, parent
def __repr__(self):
return "<Block Node: %s. Contents: %r>" % (self.name, self.nodelist)
def render(self, context):
block_context = context.render_context.get(BLOCK_CONTEXT_KEY)
with context.push():
if block_context is None:
context['block'] = self
result = self.nodelist.render(context)
else:
push = block = block_context.pop(self.name)
if block is None:
block = self
# Create new block so we can store context without thread-safety issues.
block = type(self)(block.name, block.nodelist)
block.context = context
context['block'] = block
result = block.nodelist.render(context)
if push is not None:
block_context.push(self.name, push)
return result
def super(self):
if not hasattr(self, 'context'):
raise TemplateSyntaxError(
"'%s' object has no attribute 'context'. Did you use "
"{{ block.super }} in a base template?" % self.__class__.__name__
)
render_context = self.context.render_context
if (BLOCK_CONTEXT_KEY in render_context and
render_context[BLOCK_CONTEXT_KEY].get_block(self.name) is not None):
return mark_safe(self.render(self.context))
return ''
class ExtendsNode(Node):
must_be_first = True
context_key = 'extends_context'
def __init__(self, nodelist, parent_name, template_dirs=None):
self.nodelist = nodelist
self.parent_name = parent_name
self.template_dirs = template_dirs
self.blocks = {n.name: n for n in nodelist.get_nodes_by_type(BlockNode)}
def __repr__(self):
return '<ExtendsNode: extends %s>' % self.parent_name.token
def find_template(self, template_name, context):
"""
This is a wrapper around engine.find_template(). A history is kept in
the render_context attribute between successive extends calls and
passed as the skip argument. This enables extends to work recursively
without extending the same template twice.
"""
history = context.render_context.setdefault(
self.context_key, [context.template.origin],
)
template, origin = context.template.engine.find_template(
template_name, skip=history,
)
history.append(origin)
return template
def get_parent(self, context):
parent = self.parent_name.resolve(context)
if not parent:
error_msg = "Invalid template name in 'extends' tag: %r." % parent
if self.parent_name.filters or\
isinstance(self.parent_name.var, Variable):
error_msg += " Got this from the '%s' variable." %\
self.parent_name.token
raise TemplateSyntaxError(error_msg)
if isinstance(parent, Template):
# parent is a django.template.Template
return parent
if isinstance(getattr(parent, 'template', None), Template):
# parent is a django.template.backends.django.Template
return parent.template
return self.find_template(parent, context)
def render(self, context):
compiled_parent = self.get_parent(context)
if BLOCK_CONTEXT_KEY not in context.render_context:
context.render_context[BLOCK_CONTEXT_KEY] = BlockContext()
block_context = context.render_context[BLOCK_CONTEXT_KEY]
# Add the block nodes from this node to the block context
block_context.add_blocks(self.blocks)
# If this block's parent doesn't have an extends node it is the root,
# and its block nodes also need to be added to the block context.
for node in compiled_parent.nodelist:
# The ExtendsNode has to be the first non-text node.
if not isinstance(node, TextNode):
if not isinstance(node, ExtendsNode):
blocks = {n.name: n for n in
compiled_parent.nodelist.get_nodes_by_type(BlockNode)}
block_context.add_blocks(blocks)
break
# Call Template._render explicitly so the parser context stays
# the same.
return compiled_parent._render(context)
class IncludeNode(Node):
context_key = '__include_context'
def __init__(self, template, *args, **kwargs):
self.template = template
self.extra_context = kwargs.pop('extra_context', {})
self.isolated_context = kwargs.pop('isolated_context', False)
super(IncludeNode, self).__init__(*args, **kwargs)
def render(self, context):
"""
Render the specified template and context. Cache the template object
in render_context to avoid reparsing and loading when used in a for
loop.
"""
try:
template = self.template.resolve(context)
# Does this quack like a Template?
if not callable(getattr(template, 'render', None)):
# If not, we'll try our cache, and get_template()
template_name = template
cache = context.render_context.setdefault(self.context_key, {})
template = cache.get(template_name)
if template is None:
template = context.template.engine.get_template(template_name)
cache[template_name] = template
values = {
name: var.resolve(context)
for name, var in self.extra_context.items()
}
if self.isolated_context:
return template.render(context.new(values))
with context.push(**values):
return template.render(context)
except Exception as e:
if context.template.engine.debug:
raise
template_name = getattr(context, 'template_name', None) or 'unknown'
warnings.warn(
"Rendering {%% include '%s' %%} raised %s. In Django 2.1, "
"this exception will be raised rather than silenced and "
"rendered as an empty string." %
(template_name, e.__class__.__name__),
RemovedInDjango21Warning,
)
logger.warning(
"Exception raised while rendering {%% include %%} for "
"template '%s'. Empty string rendered instead.",
template_name,
exc_info=True,
)
return ''
@register.tag('block')
def do_block(parser, token):
"""
Define a block that can be overridden by child templates.
"""
# token.split_contents() isn't useful here because this tag doesn't accept variable as arguments
bits = token.contents.split()
if len(bits) != 2:
raise TemplateSyntaxError("'%s' tag takes only one argument" % bits[0])
block_name = bits[1]
# Keep track of the names of BlockNodes found in this template, so we can
# check for duplication.
try:
if block_name in parser.__loaded_blocks:
raise TemplateSyntaxError("'%s' tag with name '%s' appears more than once" % (bits[0], block_name))
parser.__loaded_blocks.append(block_name)
except AttributeError: # parser.__loaded_blocks isn't a list yet
parser.__loaded_blocks = [block_name]
nodelist = parser.parse(('endblock',))
# This check is kept for backwards-compatibility. See #3100.
endblock = parser.next_token()
acceptable_endblocks = ('endblock', 'endblock %s' % block_name)
if endblock.contents not in acceptable_endblocks:
parser.invalid_block_tag(endblock, 'endblock', acceptable_endblocks)
return BlockNode(block_name, nodelist)
def construct_relative_path(current_template_name, relative_name):
"""
Convert a relative path (starting with './' or '../') to the full template
name based on the current_template_name.
"""
if not any(relative_name.startswith(x) for x in ["'./", "'../", '"./', '"../']):
# relative_name is a variable or a literal that doesn't contain a
# relative path.
return relative_name
new_name = posixpath.normpath(
posixpath.join(
posixpath.dirname(current_template_name.lstrip('/')),
relative_name.strip('\'"')
)
)
if new_name.startswith('../'):
raise TemplateSyntaxError(
"The relative path '%s' points outside the file hierarchy that "
"template '%s' is in." % (relative_name, current_template_name)
)
if current_template_name.lstrip('/') == new_name:
raise TemplateSyntaxError(
"The relative path '%s' was translated to template name '%s', the "
"same template in which the tag appears."
% (relative_name, current_template_name)
)
return '"%s"' % new_name
@register.tag('extends')
def do_extends(parser, token):
"""
Signal that this template extends a parent template.
This tag may be used in two ways: ``{% extends "base" %}`` (with quotes)
uses the literal value "base" as the name of the parent template to extend,
or ``{% extends variable %}`` uses the value of ``variable`` as either the
name of the parent template to extend (if it evaluates to a string) or as
the parent template itself (if it evaluates to a Template object).
"""
bits = token.split_contents()
if len(bits) != 2:
raise TemplateSyntaxError("'%s' takes one argument" % bits[0])
bits[1] = construct_relative_path(parser.origin.template_name, bits[1])
parent_name = parser.compile_filter(bits[1])
nodelist = parser.parse()
if nodelist.get_nodes_by_type(ExtendsNode):
raise TemplateSyntaxError("'%s' cannot appear more than once in the same template" % bits[0])
return ExtendsNode(nodelist, parent_name)
@register.tag('include')
def do_include(parser, token):
"""
Loads a template and renders it with the current context. You can pass
additional context using keyword arguments.
Example::
{% include "foo/some_include" %}
{% include "foo/some_include" with bar="BAZZ!" baz="BING!" %}
Use the ``only`` argument to exclude the current context when rendering
the included template::
{% include "foo/some_include" only %}
{% include "foo/some_include" with bar="1" only %}
"""
bits = token.split_contents()
if len(bits) < 2:
raise TemplateSyntaxError(
"%r tag takes at least one argument: the name of the template to "
"be included." % bits[0]
)
options = {}
remaining_bits = bits[2:]
while remaining_bits:
option = remaining_bits.pop(0)
if option in options:
raise TemplateSyntaxError('The %r option was specified more '
'than once.' % option)
if option == 'with':
value = token_kwargs(remaining_bits, parser, support_legacy=False)
if not value:
raise TemplateSyntaxError('"with" in %r tag needs at least '
'one keyword argument.' % bits[0])
elif option == 'only':
value = True
else:
raise TemplateSyntaxError('Unknown argument for %r tag: %r.' %
(bits[0], option))
options[option] = value
isolated_context = options.get('only', False)
namemap = options.get('with', {})
bits[1] = construct_relative_path(parser.origin.template_name, bits[1])
return IncludeNode(parser.compile_filter(bits[1]), extra_context=namemap,
isolated_context=isolated_context)
| mattseymour/django | django/template/loader_tags.py | Python | bsd-3-clause | 13,097 |
#!/usr/bin/env python
import argparse
def main():
parser = argparse.ArgumentParser()
parser.add_argument('--year', type=int, default=2016)
args = parser.parse_args()
if args.year == 2015:
import jsk_apc2015_common
cls_names = ['background'] + jsk_apc2015_common.get_object_list()
elif args.year == 2016:
import jsk_apc2016_common
data = jsk_apc2016_common.get_object_data()
cls_names = ['background'] + [d['name'] for d in data]
else:
raise ValueError
text = []
for cls_id, cls_name in enumerate(cls_names):
text.append('{:2}: {}'.format(cls_id, cls_name))
print('\n'.join(text))
if __name__ == '__main__':
main()
| start-jsk/jsk_apc | jsk_apc2016_common/scripts/list_objects.py | Python | bsd-3-clause | 717 |
# -*- coding: utf-8 -*-
import os
import pytest
import json
from flask import url_for
from vwadaptor.user.models import User
from vwadaptor.helpers import modelrun_serializer
from .factories import UserFactory
from .conftest import TEST_DIR
from vwpy.modelschema import load_schemas
schemas = load_schemas()
class TestModelRun:
def test_create_modelrun(self,testapp):
modelrun=dict(title="test modelrun isnobal 1",model_name="isnobal",user_id=1)
res = testapp.post_json('/api/modelruns', modelrun)
assert res.status_code == 201
assert bool(res.json['id'])
assert len(res.json['resources'])==0
def test_get_modelrun(self,testapp,modelrun):
res = testapp.get('/api/modelruns/{id}'.format(id=modelrun.id))
assert res.status_code == 200
assert res.json['id']==modelrun.id
def test_delete_modelrun(self,testapp,modelrun):
res = testapp.delete('/api/modelruns/{id}'.format(id=modelrun.id))
assert res.status_code == 204
def test_delete_modelrun(self,testapp,modelrun):
res = testapp.delete('/api/modelruns/{id}'.format(id=modelrun.id))
assert res.status_code == 204
def test_put_modelrun(self,testapp,modelrun):
new_title="Changed Title"
res = testapp.put_json('/api/modelruns/{id}'.format(id=modelrun.id),{'title':new_title})
assert res.status_code == 200
assert res.json['title'] == new_title
def test_upload_resource(self,testapp,modelrun):
resource_name = 'isnobal.nc'
files = [('file',os.path.join(TEST_DIR,'data/'+resource_name))]
res = testapp.post('/api/modelruns/{id}/upload'.format(id=modelrun.id),params=[('resource_type','input')],upload_files=files)
assert res.status_code == 201
assert res.json['resource']['resource_name'] == resource_name
assert resource_name in res.json['resource']['resource_url']
def test_start_modelrun_fail(self,testapp,modelrun):
res = testapp.put('/api/modelruns/{id}/start'.format(id=modelrun.id),expect_errors=True)
assert res.status_code == 400
assert set(res.json['missing']) <= set(schemas[modelrun.model_name]['resources']['inputs'].keys())
def test_start_modelrun_pass(self,testapp,modelrun):
resource_name = 'isnobal.nc'
files = [('file',os.path.join(TEST_DIR,'data/'+resource_name))]
res = testapp.post('/api/modelruns/{id}/upload'.format(id=modelrun.id),params=[('resource_type','input')],upload_files=files)
assert res.status_code == 201
res = testapp.put('/api/modelruns/{id}/start'.format(id=modelrun.id))
assert res.status_code == 200
| itsrifat/vwadaptor | tests/test_api.py | Python | bsd-3-clause | 2,675 |
# Generated by Django 2.0.4 on 2018-05-04 17:13
from django.db import migrations, models
class Migration(migrations.Migration):
dependencies = [
("wagtaildocs", "0007_merge"),
]
operations = [
migrations.AddField(
model_name="document",
name="file_size",
field=models.PositiveIntegerField(editable=False, null=True),
),
]
| rsalmaso/wagtail | wagtail/documents/migrations/0008_document_file_size.py | Python | bsd-3-clause | 403 |
# -*- coding: utf-8 -*-
import doctest
import logging
import os
from unittest.mock import Mock, patch, call
from uuid import uuid4
from django.test import TransactionTestCase
from eventkit_cloud.utils import geopackage
from eventkit_cloud.utils.geopackage import (
add_geojson_to_geopackage,
get_table_count,
get_table_names,
get_tile_table_names,
get_table_gpkg_contents_information,
set_gpkg_contents_bounds,
get_zoom_levels_table,
remove_empty_zoom_levels,
remove_zoom_level,
get_tile_matrix_table_zoom_levels,
check_content_exists,
check_zoom_levels,
get_table_info,
create_table_from_existing,
create_metadata_tables,
create_extension_table,
add_file_metadata,
)
logger = logging.getLogger(__name__)
def load_tests(loader, tests, ignore):
tests.addTests(doctest.DocTestSuite(geopackage))
return tests
class TestGeopackage(TransactionTestCase):
def setUp(self):
self.path = os.path.dirname(os.path.realpath(__file__))
self.task_uid = uuid4()
@patch("eventkit_cloud.utils.geopackage.sqlite3")
def test_get_table_count(self, sqlite3):
expected_count = 4
gpkg = "/test/file.gpkg"
cursor_mock = Mock()
cursor_mock.fetchone.return_value = [expected_count]
sqlite3.connect().__enter__().execute.return_value = cursor_mock
bad_table_name = "test;this"
returned_count = get_table_count(gpkg, bad_table_name)
sqlite3.connect().__enter__().execute.assert_not_called()
self.assertFalse(returned_count)
table_name = "test"
returned_count = get_table_count(gpkg, table_name)
sqlite3.connect().__enter__().execute.assert_called_once_with("SELECT COUNT(*) FROM '{0}';".format(table_name))
self.assertEqual(expected_count, returned_count)
@patch("eventkit_cloud.utils.geopackage.sqlite3")
def test_get_table_names(self, sqlite3):
expected_table_names = ["test1", "test2"]
mock_table_names = [(expected_table_names[0],), (expected_table_names[1],)]
gpkg = "/test/file.gpkg"
sqlite3.connect().__enter__().execute.return_value = mock_table_names
return_table_names = get_table_names(gpkg)
sqlite3.connect().__enter__().execute.assert_called_once_with("SELECT table_name FROM gpkg_contents;")
self.assertEqual(expected_table_names, return_table_names)
@patch("eventkit_cloud.utils.geopackage.sqlite3")
def test_get_tile_table_names(self, sqlite3):
expected_table_names = ["test1", "test2"]
mock_table_names = [(expected_table_names[0],), (expected_table_names[1],)]
gpkg = "/test/file.gpkg"
sqlite3.connect().__enter__().execute.return_value = mock_table_names
return_table_names = get_tile_table_names(gpkg)
sqlite3.connect().__enter__().execute.assert_called_once_with(
"SELECT table_name FROM gpkg_contents WHERE data_type = 'tiles';"
)
self.assertEqual(expected_table_names, return_table_names)
@patch("eventkit_cloud.utils.geopackage.sqlite3")
def test_set_gpkg_contents_bounds(self, sqlite3):
table_name = "test1"
gpkg = "/test/file.gpkg"
bbox = [-1, 0, 2, 1]
sqlite3.connect().__enter__().execute.return_value = Mock(rowcount=1)
set_gpkg_contents_bounds(gpkg, table_name, bbox)
sqlite3.connect().__enter__().execute.assert_called_once_with(
"UPDATE gpkg_contents SET min_x = {0}, min_y = {1}, max_x = {2}, max_y = {3} "
"WHERE table_name = '{4}';".format(bbox[0], bbox[1], bbox[2], bbox[3], table_name)
)
with self.assertRaises(Exception):
sqlite3.connect().__enter__().execute.return_value = Mock(rowcount=0)
set_gpkg_contents_bounds(gpkg, table_name, bbox)
@patch("eventkit_cloud.utils.geopackage.sqlite3")
def test_get_zoom_levels_table(self, sqlite3):
expected_zoom_levels = [0, 1, 2]
mock_zoom_levels = [(expected_zoom_levels[0],), (expected_zoom_levels[1],), (expected_zoom_levels[2],)]
gpkg = "/test/file.gpkg"
sqlite3.connect().__enter__().execute.return_value = mock_zoom_levels
bad_table_name = "test;this"
returned_zoom_levels = get_zoom_levels_table(gpkg, bad_table_name)
sqlite3.connect().__enter__().execute.assert_not_called()
self.assertFalse(returned_zoom_levels)
table_name = "test"
returned_zoom_levels = get_zoom_levels_table(gpkg, table_name)
sqlite3.connect().__enter__().execute.assert_called_once_with(
"SELECT DISTINCT zoom_level FROM '{0}';".format(table_name)
)
self.assertEqual(expected_zoom_levels, returned_zoom_levels)
@patch("eventkit_cloud.utils.geopackage.sqlite3")
def test_remove_zoom_level(self, sqlite3):
zoom_level = 1
gpkg = "/test/file.gpkg"
with self.assertRaises(Exception):
bad_table_name = "test;this"
remove_zoom_level(gpkg, bad_table_name, zoom_level)
table_name = "test"
with self.assertRaises(Exception):
sqlite3.connect().__enter__().execute.return_value = Mock(rowcount=0)
remove_zoom_level(gpkg, table_name, zoom_level)
sqlite3.reset_mock()
sqlite3.connect().__enter__().execute.return_value = Mock(rowcount=1)
remove_zoom_level(gpkg, table_name, zoom_level)
sqlite3.connect().__enter__().execute.assert_called_once_with(
"DELETE FROM gpkg_tile_matrix WHERE table_name = '{0}' AND zoom_level = '{1}';".format(
table_name, int(zoom_level)
)
)
@patch("eventkit_cloud.utils.geopackage.sqlite3")
def test_get_tile_matrix_table_zoom_levels(self, sqlite3):
expected_zoom_levels = [0, 1, 2]
mock_zoom_levels = [(expected_zoom_levels[0],), (expected_zoom_levels[1],), (expected_zoom_levels[2],)]
gpkg = "/test/file.gpkg"
sqlite3.connect().__enter__().execute.return_value = mock_zoom_levels
bad_table_name = "test;this"
returned_zoom_levels = get_tile_matrix_table_zoom_levels(gpkg, bad_table_name)
sqlite3.connect().__enter__().execute.assert_not_called()
self.assertFalse(returned_zoom_levels)
table_name = "test"
returned_zoom_levels = get_tile_matrix_table_zoom_levels(gpkg, table_name)
sqlite3.connect().__enter__().execute.assert_called_once_with(
"SELECT zoom_level FROM gpkg_tile_matrix WHERE table_name = '{0}';".format(table_name)
)
self.assertEqual(expected_zoom_levels, returned_zoom_levels)
@patch("eventkit_cloud.utils.geopackage.remove_zoom_level")
@patch("eventkit_cloud.utils.geopackage.get_tile_matrix_table_zoom_levels")
@patch("eventkit_cloud.utils.geopackage.get_zoom_levels_table")
@patch("eventkit_cloud.utils.geopackage.get_tile_table_names")
def test_remove_empty_zoom_levels(
self, get_tile_table_names, get_zoom_levels_table, get_tile_matrix_table_zoom_levels, remove_zoom_level
):
gpkg = "/test/file.gpkg"
table_names = ["test_1", "test_2"]
populated_zoom_levels = [1, 2, 3]
tile_matrix_zoom_levels = [1, 2, 3, 4]
get_tile_table_names.return_value = table_names
get_zoom_levels_table.return_value = populated_zoom_levels
get_tile_matrix_table_zoom_levels.return_value = tile_matrix_zoom_levels
remove_empty_zoom_levels(gpkg)
get_tile_table_names.assert_called_once_with(gpkg)
self.assertEqual([call(gpkg, table_names[0]), call(gpkg, table_names[1])], get_zoom_levels_table.mock_calls)
self.assertEqual(
[call(gpkg, table_names[0]), call(gpkg, table_names[1])], get_tile_matrix_table_zoom_levels.mock_calls
)
self.assertEqual([call(gpkg, table_names[0], 4), call(gpkg, table_names[1], 4)], remove_zoom_level.mock_calls)
@patch("eventkit_cloud.utils.geopackage.get_table_count")
@patch("eventkit_cloud.utils.geopackage.get_table_names")
def test_check_content_exists(self, get_table_names, get_table_count):
gpkg = "/test/file.gpkg"
table_names = ["test_1", "test_2"]
get_table_names.return_value = table_names
get_table_count.return_value = 0
returned_value = check_content_exists(gpkg)
self.assertFalse(returned_value)
get_table_count.return_value = 1
returned_value = check_content_exists(gpkg)
self.assertTrue(returned_value)
self.assertEqual([call(gpkg), call(gpkg)], get_table_names.mock_calls)
@patch("eventkit_cloud.utils.geopackage.get_zoom_levels_table")
@patch("eventkit_cloud.utils.geopackage.get_tile_matrix_table_zoom_levels")
@patch("eventkit_cloud.utils.geopackage.get_table_names")
def test_check_zoom_levels(self, get_table_names, get_tile_matrix_table_zoom_levels, get_zoom_levels_table):
gpkg = "/test/file.gpkg"
table_names = ["test_1", "test_2"]
get_table_names.return_value = table_names
get_tile_matrix_table_zoom_levels.return_value = [0, 1, 2, 3]
get_zoom_levels_table.return_value = [0, 1, 2]
returned_value = check_zoom_levels(gpkg)
self.assertFalse(returned_value)
get_tile_matrix_table_zoom_levels.return_value = [0, 1, 2]
get_zoom_levels_table.return_value = [0, 1, 2]
returned_value = check_zoom_levels(gpkg)
self.assertTrue(returned_value)
self.assertEqual([call(gpkg), call(gpkg)], get_table_names.mock_calls)
@patch("eventkit_cloud.utils.gdalutils.convert")
@patch("builtins.open")
def test_add_geojson_to_geopackage(self, open, mock_convert):
geojson = "{}"
gpkg = None
with self.assertRaises(Exception):
add_geojson_to_geopackage(geojson=geojson, gpkg=gpkg)
geojson = "{}"
gpkg = "test.gpkg"
layer_name = "test_layer"
add_geojson_to_geopackage(geojson=geojson, gpkg=gpkg, layer_name=layer_name, task_uid=self.task_uid)
open.assert_called_once_with(
os.path.join(os.path.dirname(gpkg), "{0}.geojson".format(os.path.splitext(os.path.basename(gpkg))[0])), "w"
)
gdal_mock = mock_convert.return_value
gdal_mock.convert.called_once_with(
driver="gpkg", input_file=geojson, output_file=gpkg, creation_options="-nln {0}".format(layer_name)
)
@patch("eventkit_cloud.utils.geopackage.sqlite3")
@patch("eventkit_cloud.utils.geopackage.get_table_info")
def test_create_table_from_existing(self, mock_table_info, mock_sqlite3):
table_info = [(1, "col1", "INT", 0, 0, 1), (1, "col2", "INT", 0, 0, 0)]
mock_table_info.return_value = table_info
gpkg = "test.gpkg"
old_table = "old"
new_table = "new"
create_table_from_existing(gpkg, old_table, new_table)
mock_table_info.assert_called_once_with(gpkg, old_table)
mock_sqlite3.connect().__enter__().execute.assert_called_once_with(
"CREATE TABLE {0} (col1 INTEGER PRIMARY KEY AUTOINCREMENT,col2 INT);".format(new_table)
)
@patch("eventkit_cloud.utils.geopackage.sqlite3")
def test_get_table_info(self, mock_sqlite3):
gpkg = "test.gpkg"
table = "table"
expected_response = [(1, "col1", "INT", 0, 0, 1), (1, "col2", "INT", 0, 0, 0)]
mock_sqlite3.connect().__enter__().execute.return_value = expected_response
response = get_table_info(gpkg, table)
mock_sqlite3.connect().__enter__().execute.assert_called_once_with("PRAGMA table_info({0});".format(table))
self.assertEqual(expected_response, response)
@patch("eventkit_cloud.utils.geopackage.sqlite3")
def test_get_table_gpkg_contents_information(self, mock_sqlite3):
gpkg = "test.gpkg"
table = "table"
query_response = (table, "tiles", "identifier", "description", "datetime", -180, -90, 190, 90, 4326)
expected_response = {
"table_name": query_response[0],
"data_type": query_response[1],
"identifier": query_response[2],
"description": query_response[3],
"last_change": query_response[4],
"min_x": query_response[5],
"min_y": query_response[6],
"max_x": query_response[7],
"max_y": query_response[8],
"srs_id": query_response[9],
}
mock_sqlite3.connect().__enter__().execute().fetchone.return_value = query_response
response = get_table_gpkg_contents_information(gpkg, table)
mock_sqlite3.connect().__enter__().execute.assert_called_with(
"SELECT table_name, data_type, identifier, description, last_change, min_x, min_y, max_x, max_y, srs_id "
"FROM gpkg_contents WHERE table_name = '{0}';".format(table)
)
self.assertEqual(expected_response, response)
@patch("eventkit_cloud.utils.geopackage.sqlite3")
def test_create_extension_table(self, mock_sqlite3):
gpkg = "test.gpkg"
create_extension_table(gpkg)
mock_sqlite3.connect().__enter__().execute.assert_called_once()
@patch("eventkit_cloud.utils.geopackage.create_extension_table")
@patch("eventkit_cloud.utils.geopackage.sqlite3")
def test_create_metadata_tables(self, mock_sqlite3, mock_create_extension_table):
gpkg = "test.gpkg"
create_metadata_tables(gpkg)
mock_sqlite3.connect().__enter__().execute.assert_called()
mock_create_extension_table.assert_called_once_with(gpkg)
@patch("eventkit_cloud.utils.geopackage.create_metadata_tables")
@patch("eventkit_cloud.utils.geopackage.sqlite3")
def test_add_file_metadata(self, mock_sqlite3, mock_create_metadata_tables):
gpkg = "test.gpkg"
metadata = "sample data"
add_file_metadata(gpkg, metadata)
mock_sqlite3.connect().__enter__().execute.assert_called()
mock_create_metadata_tables.assert_called_once_with(gpkg)
| venicegeo/eventkit-cloud | eventkit_cloud/utils/tests/test_geopackage.py | Python | bsd-3-clause | 14,062 |
from __future__ import absolute_import, print_function, division
import numpy
from nose.plugins.skip import SkipTest
import theano
from theano import tensor
import theano.tests.unittest_tools as utt
import theano.tensor.nnet.tests.test_blocksparse
import theano.sandbox.cuda as cuda_ndarray
from theano.sandbox.cuda.blocksparse import (GpuSparseBlockGemv,
GpuSparseBlockOuter,
gpu_sparse_block_gemv,
gpu_sparse_block_outer)
from theano.sandbox.cuda.var import float32_shared_constructor
if not cuda_ndarray.cuda_available:
raise SkipTest('Optional package cuda disabled')
if theano.config.mode == 'FAST_COMPILE':
mode_with_gpu = theano.compile.mode.get_mode('FAST_RUN').including('gpu')
else:
mode_with_gpu = theano.compile.mode.get_default_mode().including('gpu')
class BlockSparse_Gemv_and_Outer(
theano.tensor.nnet.tests.test_blocksparse.BlockSparse_Gemv_and_Outer):
def setUp(self):
utt.seed_rng()
self.mode = mode_with_gpu.excluding('constant_folding')
self.gemv_op = gpu_sparse_block_gemv
self.outer_op = gpu_sparse_block_outer
self.gemv_class = GpuSparseBlockGemv
self.outer_class = GpuSparseBlockOuter
# This test is temporarily disabled since we disabled the output_merge
# and alpha_merge optimizations for blocksparse due to brokeness.
# Re-enable when those are re-added.
def Xtest_blocksparse_grad_merge(self):
b = tensor.fmatrix()
h = tensor.ftensor3()
iIdx = tensor.lmatrix()
oIdx = tensor.lmatrix()
W_val, h_val, iIdx_val, b_val, oIdx_val = self.gemv_data()
W = float32_shared_constructor(W_val)
o = gpu_sparse_block_gemv(b.take(oIdx, axis=0), W, h, iIdx, oIdx)
gW = theano.grad(o.sum(), W)
lr = numpy.asarray(0.05, dtype='float32')
upd = W - lr * gW
f1 = theano.function([h, iIdx, b, oIdx], updates=[(W, upd)],
mode=mode_with_gpu)
# Make sure the lr update was merged.
assert isinstance(f1.maker.fgraph.outputs[0].owner.op,
GpuSparseBlockOuter)
# Exclude the merge optimizations.
mode = mode_with_gpu.excluding('local_merge_blocksparse_alpha')
mode = mode.excluding('local_merge_blocksparse_output')
f2 = theano.function([h, iIdx, b, oIdx], updates=[(W, upd)], mode=mode)
# Make sure the lr update is not merged.
assert not isinstance(f2.maker.fgraph.outputs[0].owner.op,
GpuSparseBlockOuter)
f2(h_val, iIdx_val, b_val, oIdx_val)
W_ref = W.get_value()
# reset the var
W.set_value(W_val)
f1(h_val, iIdx_val, b_val, oIdx_val)
W_opt = W.get_value()
utt.assert_allclose(W_ref, W_opt)
| JazzeYoung/VeryDeepAutoEncoder | theano/sandbox/cuda/tests/test_blocksparse.py | Python | bsd-3-clause | 2,932 |
# Copyright (c) 2012, GPy authors (see AUTHORS.txt).
# Licensed under the BSD 3-clause license (see LICENSE.txt)
from .kern import Kern
from ...core.parameterization import Param
from paramz.transformations import Logexp
import numpy as np
from ...util.multioutput import index_to_slices
class ODE_st(Kern):
"""
kernel resultiong from a first order ODE with OU driving GP
:param input_dim: the number of input dimension, has to be equal to one
:type input_dim: int
:param varianceU: variance of the driving GP
:type varianceU: float
:param lengthscaleU: lengthscale of the driving GP (sqrt(3)/lengthscaleU)
:type lengthscaleU: float
:param varianceY: 'variance' of the transfer function
:type varianceY: float
:param lengthscaleY: 'lengthscale' of the transfer function (1/lengthscaleY)
:type lengthscaleY: float
:rtype: kernel object
"""
def __init__(self, input_dim, a=1.,b=1., c=1.,variance_Yx=3.,variance_Yt=1.5, lengthscale_Yx=1.5, lengthscale_Yt=1.5, active_dims=None, name='ode_st'):
assert input_dim ==3, "only defined for 3 input dims"
super(ODE_st, self).__init__(input_dim, active_dims, name)
self.variance_Yt = Param('variance_Yt', variance_Yt, Logexp())
self.variance_Yx = Param('variance_Yx', variance_Yx, Logexp())
self.lengthscale_Yt = Param('lengthscale_Yt', lengthscale_Yt, Logexp())
self.lengthscale_Yx = Param('lengthscale_Yx', lengthscale_Yx, Logexp())
self.a= Param('a', a, Logexp())
self.b = Param('b', b, Logexp())
self.c = Param('c', c, Logexp())
self.link_parameters(self.a, self.b, self.c, self.variance_Yt, self.variance_Yx, self.lengthscale_Yt,self.lengthscale_Yx)
def K(self, X, X2=None):
# model : -a d^2y/dx^2 + b dy/dt + c * y = U
# kernel Kyy rbf spatiol temporal
# vyt Y temporal variance vyx Y spatiol variance lyt Y temporal lengthscale lyx Y spatiol lengthscale
# kernel Kuu doper( doper(Kyy))
# a b c lyt lyx vyx*vyt
"""Compute the covariance matrix between X and X2."""
X,slices = X[:,:-1],index_to_slices(X[:,-1])
if X2 is None:
X2,slices2 = X,slices
K = np.zeros((X.shape[0], X.shape[0]))
else:
X2,slices2 = X2[:,:-1],index_to_slices(X2[:,-1])
K = np.zeros((X.shape[0], X2.shape[0]))
tdist = (X[:,0][:,None] - X2[:,0][None,:])**2
xdist = (X[:,1][:,None] - X2[:,1][None,:])**2
ttdist = (X[:,0][:,None] - X2[:,0][None,:])
#rdist = [tdist,xdist]
#dist = np.abs(X - X2.T)
vyt = self.variance_Yt
vyx = self.variance_Yx
lyt=1/(2*self.lengthscale_Yt)
lyx=1/(2*self.lengthscale_Yx)
a = self.a ## -a is used in the model, negtive diffusion
b = self.b
c = self.c
kyy = lambda tdist,xdist: np.exp(-lyt*(tdist) -lyx*(xdist))
k1 = lambda tdist: (2*lyt - 4*lyt**2 * (tdist) )
k2 = lambda xdist: ( 4*lyx**2 * (xdist) - 2*lyx )
k3 = lambda xdist: ( 3*4*lyx**2 - 6*8*xdist*lyx**3 + 16*xdist**2*lyx**4 )
k4 = lambda ttdist: 2*lyt*(ttdist)
for i, s1 in enumerate(slices):
for j, s2 in enumerate(slices2):
for ss1 in s1:
for ss2 in s2:
if i==0 and j==0:
K[ss1,ss2] = vyt*vyx*kyy(tdist[ss1,ss2],xdist[ss1,ss2])
elif i==0 and j==1:
K[ss1,ss2] = (-a*k2(xdist[ss1,ss2]) + b*k4(ttdist[ss1,ss2]) + c)*vyt*vyx*kyy(tdist[ss1,ss2],xdist[ss1,ss2])
#K[ss1,ss2]= np.where( rdist[ss1,ss2]>0 , kuyp(np.abs(rdist[ss1,ss2])), kuyn(np.abs(rdist[ss1,ss2]) ) )
#K[ss1,ss2]= np.where( rdist[ss1,ss2]>0 , kuyp(rdist[ss1,ss2]), kuyn(rdist[ss1,ss2] ) )
elif i==1 and j==1:
K[ss1,ss2] = ( b**2*k1(tdist[ss1,ss2]) - 2*a*c*k2(xdist[ss1,ss2]) + a**2*k3(xdist[ss1,ss2]) + c**2 )* vyt*vyx* kyy(tdist[ss1,ss2],xdist[ss1,ss2])
else:
K[ss1,ss2] = (-a*k2(xdist[ss1,ss2]) - b*k4(ttdist[ss1,ss2]) + c)*vyt*vyx*kyy(tdist[ss1,ss2],xdist[ss1,ss2])
#K[ss1,ss2]= np.where( rdist[ss1,ss2]>0 , kyup(np.abs(rdist[ss1,ss2])), kyun(np.abs(rdist[ss1,ss2]) ) )
#K[ss1,ss2] = np.where( rdist[ss1,ss2]>0 , kyup(rdist[ss1,ss2]), kyun(rdist[ss1,ss2] ) )
#stop
return K
def Kdiag(self, X):
"""Compute the diagonal of the covariance matrix associated to X."""
vyt = self.variance_Yt
vyx = self.variance_Yx
lyt = 1./(2*self.lengthscale_Yt)
lyx = 1./(2*self.lengthscale_Yx)
a = self.a
b = self.b
c = self.c
## dk^2/dtdt'
k1 = (2*lyt )*vyt*vyx
## dk^2/dx^2
k2 = ( - 2*lyx )*vyt*vyx
## dk^4/dx^2dx'^2
k3 = ( 4*3*lyx**2 )*vyt*vyx
Kdiag = np.zeros(X.shape[0])
slices = index_to_slices(X[:,-1])
for i, ss1 in enumerate(slices):
for s1 in ss1:
if i==0:
Kdiag[s1]+= vyt*vyx
elif i==1:
#i=1
Kdiag[s1]+= b**2*k1 - 2*a*c*k2 + a**2*k3 + c**2*vyt*vyx
#Kdiag[s1]+= Vu*Vy*(k1+k2+k3)
else:
raise ValueError("invalid input/output index")
return Kdiag
def update_gradients_full(self, dL_dK, X, X2=None):
#def dK_dtheta(self, dL_dK, X, X2, target):
"""derivative of the covariance matrix with respect to the parameters."""
X,slices = X[:,:-1],index_to_slices(X[:,-1])
if X2 is None:
X2,slices2 = X,slices
K = np.zeros((X.shape[0], X.shape[0]))
else:
X2,slices2 = X2[:,:-1],index_to_slices(X2[:,-1])
vyt = self.variance_Yt
vyx = self.variance_Yx
lyt = 1./(2*self.lengthscale_Yt)
lyx = 1./(2*self.lengthscale_Yx)
a = self.a
b = self.b
c = self.c
tdist = (X[:,0][:,None] - X2[:,0][None,:])**2
xdist = (X[:,1][:,None] - X2[:,1][None,:])**2
#rdist = [tdist,xdist]
ttdist = (X[:,0][:,None] - X2[:,0][None,:])
rd=tdist.shape[0]
dka = np.zeros([rd,rd])
dkb = np.zeros([rd,rd])
dkc = np.zeros([rd,rd])
dkYdvart = np.zeros([rd,rd])
dkYdvarx = np.zeros([rd,rd])
dkYdlent = np.zeros([rd,rd])
dkYdlenx = np.zeros([rd,rd])
kyy = lambda tdist,xdist: np.exp(-lyt*(tdist) -lyx*(xdist))
#k1 = lambda tdist: (lyt - lyt**2 * (tdist) )
#k2 = lambda xdist: ( lyx**2 * (xdist) - lyx )
#k3 = lambda xdist: ( 3*lyx**2 - 6*xdist*lyx**3 + xdist**2*lyx**4 )
#k4 = lambda tdist: -lyt*np.sqrt(tdist)
k1 = lambda tdist: (2*lyt - 4*lyt**2 * (tdist) )
k2 = lambda xdist: ( 4*lyx**2 * (xdist) - 2*lyx )
k3 = lambda xdist: ( 3*4*lyx**2 - 6*8*xdist*lyx**3 + 16*xdist**2*lyx**4 )
k4 = lambda ttdist: 2*lyt*(ttdist)
dkyydlyx = lambda tdist,xdist: kyy(tdist,xdist)*(-xdist)
dkyydlyt = lambda tdist,xdist: kyy(tdist,xdist)*(-tdist)
dk1dlyt = lambda tdist: 2. - 4*2.*lyt*tdist
dk2dlyx = lambda xdist: (4.*2.*lyx*xdist -2.)
dk3dlyx = lambda xdist: (6.*4.*lyx - 18.*8*xdist*lyx**2 + 4*16*xdist**2*lyx**3)
dk4dlyt = lambda ttdist: 2*(ttdist)
for i, s1 in enumerate(slices):
for j, s2 in enumerate(slices2):
for ss1 in s1:
for ss2 in s2:
if i==0 and j==0:
dka[ss1,ss2] = 0
dkb[ss1,ss2] = 0
dkc[ss1,ss2] = 0
dkYdvart[ss1,ss2] = vyx*kyy(tdist[ss1,ss2],xdist[ss1,ss2])
dkYdvarx[ss1,ss2] = vyt*kyy(tdist[ss1,ss2],xdist[ss1,ss2])
dkYdlenx[ss1,ss2] = vyt*vyx*dkyydlyx(tdist[ss1,ss2],xdist[ss1,ss2])
dkYdlent[ss1,ss2] = vyt*vyx*dkyydlyt(tdist[ss1,ss2],xdist[ss1,ss2])
elif i==0 and j==1:
dka[ss1,ss2] = -k2(xdist[ss1,ss2])*vyt*vyx*kyy(tdist[ss1,ss2],xdist[ss1,ss2])
dkb[ss1,ss2] = k4(ttdist[ss1,ss2])*vyt*vyx*kyy(tdist[ss1,ss2],xdist[ss1,ss2])
dkc[ss1,ss2] = vyt*vyx*kyy(tdist[ss1,ss2],xdist[ss1,ss2])
#dkYdvart[ss1,ss2] = 0
#dkYdvarx[ss1,ss2] = 0
#dkYdlent[ss1,ss2] = 0
#dkYdlenx[ss1,ss2] = 0
dkYdvart[ss1,ss2] = (-a*k2(xdist[ss1,ss2])+b*k4(ttdist[ss1,ss2])+c)*vyx*kyy(tdist[ss1,ss2],xdist[ss1,ss2])
dkYdvarx[ss1,ss2] = (-a*k2(xdist[ss1,ss2])+b*k4(ttdist[ss1,ss2])+c)*vyt*kyy(tdist[ss1,ss2],xdist[ss1,ss2])
dkYdlent[ss1,ss2] = vyt*vyx*dkyydlyt(tdist[ss1,ss2],xdist[ss1,ss2])* (-a*k2(xdist[ss1,ss2])+b*k4(ttdist[ss1,ss2])+c)+\
vyt*vyx*kyy(tdist[ss1,ss2],xdist[ss1,ss2])*b*dk4dlyt(ttdist[ss1,ss2])
dkYdlenx[ss1,ss2] = vyt*vyx*dkyydlyx(tdist[ss1,ss2],xdist[ss1,ss2])*(-a*k2(xdist[ss1,ss2])+b*k4(ttdist[ss1,ss2])+c)+\
vyt*vyx*kyy(tdist[ss1,ss2],xdist[ss1,ss2])*(-a*dk2dlyx(xdist[ss1,ss2]))
elif i==1 and j==1:
dka[ss1,ss2] = (2*a*k3(xdist[ss1,ss2]) - 2*c*k2(xdist[ss1,ss2]))*vyt*vyx* kyy(tdist[ss1,ss2],xdist[ss1,ss2])
dkb[ss1,ss2] = 2*b*k1(tdist[ss1,ss2])*vyt*vyx* kyy(tdist[ss1,ss2],xdist[ss1,ss2])
dkc[ss1,ss2] = (-2*a*k2(xdist[ss1,ss2]) + 2*c )*vyt*vyx* kyy(tdist[ss1,ss2],xdist[ss1,ss2])
dkYdvart[ss1,ss2] = ( b**2*k1(tdist[ss1,ss2]) - 2*a*c*k2(xdist[ss1,ss2]) + a**2*k3(xdist[ss1,ss2]) + c**2 )*vyx* kyy(tdist[ss1,ss2],xdist[ss1,ss2])
dkYdvarx[ss1,ss2] = ( b**2*k1(tdist[ss1,ss2]) - 2*a*c*k2(xdist[ss1,ss2]) + a**2*k3(xdist[ss1,ss2]) + c**2 )*vyt* kyy(tdist[ss1,ss2],xdist[ss1,ss2])
dkYdlent[ss1,ss2] = vyt*vyx*dkyydlyt(tdist[ss1,ss2],xdist[ss1,ss2])*( b**2*k1(tdist[ss1,ss2]) - 2*a*c*k2(xdist[ss1,ss2]) + a**2*k3(xdist[ss1,ss2]) + c**2 ) +\
vyx*vyt*kyy(tdist[ss1,ss2],xdist[ss1,ss2])*b**2*dk1dlyt(tdist[ss1,ss2])
dkYdlenx[ss1,ss2] = vyt*vyx*dkyydlyx(tdist[ss1,ss2],xdist[ss1,ss2])*( b**2*k1(tdist[ss1,ss2]) - 2*a*c*k2(xdist[ss1,ss2]) + a**2*k3(xdist[ss1,ss2]) + c**2 ) +\
vyx*vyt*kyy(tdist[ss1,ss2],xdist[ss1,ss2])* (-2*a*c*dk2dlyx(xdist[ss1,ss2]) + a**2*dk3dlyx(xdist[ss1,ss2]) )
else:
dka[ss1,ss2] = -k2(xdist[ss1,ss2])*vyt*vyx*kyy(tdist[ss1,ss2],xdist[ss1,ss2])
dkb[ss1,ss2] = -k4(ttdist[ss1,ss2])*vyt*vyx*kyy(tdist[ss1,ss2],xdist[ss1,ss2])
dkc[ss1,ss2] = vyt*vyx*kyy(tdist[ss1,ss2],xdist[ss1,ss2])
#dkYdvart[ss1,ss2] = 0
#dkYdvarx[ss1,ss2] = 0
#dkYdlent[ss1,ss2] = 0
#dkYdlenx[ss1,ss2] = 0
dkYdvart[ss1,ss2] = (-a*k2(xdist[ss1,ss2])-b*k4(ttdist[ss1,ss2])+c)*vyx*kyy(tdist[ss1,ss2],xdist[ss1,ss2])
dkYdvarx[ss1,ss2] = (-a*k2(xdist[ss1,ss2])-b*k4(ttdist[ss1,ss2])+c)*vyt*kyy(tdist[ss1,ss2],xdist[ss1,ss2])
dkYdlent[ss1,ss2] = vyt*vyx*dkyydlyt(tdist[ss1,ss2],xdist[ss1,ss2])* (-a*k2(xdist[ss1,ss2])-b*k4(ttdist[ss1,ss2])+c)+\
vyt*vyx*kyy(tdist[ss1,ss2],xdist[ss1,ss2])*(-1)*b*dk4dlyt(ttdist[ss1,ss2])
dkYdlenx[ss1,ss2] = vyt*vyx*dkyydlyx(tdist[ss1,ss2],xdist[ss1,ss2])*(-a*k2(xdist[ss1,ss2])-b*k4(ttdist[ss1,ss2])+c)+\
vyt*vyx*kyy(tdist[ss1,ss2],xdist[ss1,ss2])*(-a*dk2dlyx(xdist[ss1,ss2]))
self.a.gradient = np.sum(dka * dL_dK)
self.b.gradient = np.sum(dkb * dL_dK)
self.c.gradient = np.sum(dkc * dL_dK)
self.variance_Yt.gradient = np.sum(dkYdvart * dL_dK) # Vy
self.variance_Yx.gradient = np.sum(dkYdvarx * dL_dK)
self.lengthscale_Yt.gradient = np.sum(dkYdlent*(-0.5*self.lengthscale_Yt**(-2)) * dL_dK) #ly np.sum(dktheta2*(-self.lengthscale_Y**(-2)) * dL_dK)
self.lengthscale_Yx.gradient = np.sum(dkYdlenx*(-0.5*self.lengthscale_Yx**(-2)) * dL_dK)
| SheffieldML/GPy | GPy/kern/src/ODE_st.py | Python | bsd-3-clause | 12,839 |
import pytest
from django.template.loader import render_to_string
from tests.thumbnail_tests.utils import BaseTestCase
pytestmark = pytest.mark.django_db
class FilterTestCase(BaseTestCase):
def test_html_filter(self):
text = '<img alt="A image!" src="http://dummyimage.com/800x800" />'
val = render_to_string('htmlfilter.html', {'text': text, }).strip()
self.assertEqual(
'<img alt="A image!" '
'src="/media/test/cache/2e/35/2e3517d8aa949728b1ee8b26c5a7bbc4.jpg" />',
val
)
def test_html_filter_local_url(self):
text = '<img alt="A image!" src="/media/500x500.jpg" />'
val = render_to_string('htmlfilter.html', {'text': text, }).strip()
self.assertEqual(
'<img alt="A image!" '
'src="/media/test/cache/c7/f2/c7f2880b48e9f07d46a05472c22f0fde.jpg" />',
val
)
def test_markdown_filter(self):
text = '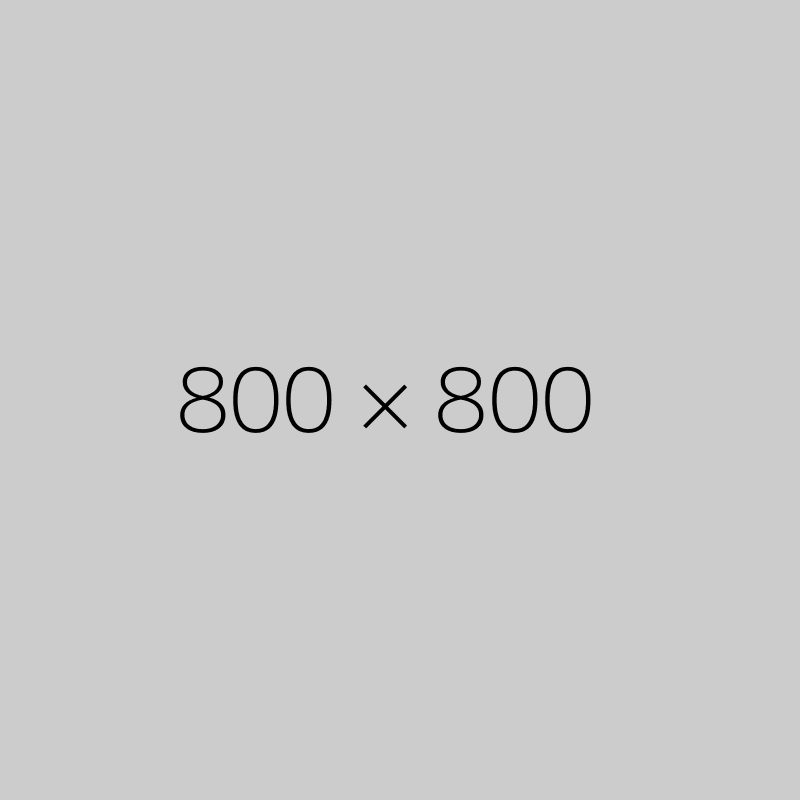'
val = render_to_string('markdownfilter.html', {'text': text, }).strip()
self.assertEqual(
'',
val
)
def test_markdown_filter_local_url(self):
text = ''
val = render_to_string('markdownfilter.html', {'text': text, }).strip()
self.assertEqual(
'',
val
)
| mariocesar/sorl-thumbnail | tests/thumbnail_tests/test_filters.py | Python | bsd-3-clause | 1,546 |
"""
Integrate functions by rewriting them as Meijer G-functions.
There are three user-visible functions that can be used by other parts of the
sympy library to solve various integration problems:
- meijerint_indefinite
- meijerint_definite
- meijerint_inversion
They can be used to compute, respectively, indefinite integrals, definite
integrals over intervals of the real line, and inverse laplace-type integrals
(from c-I*oo to c+I*oo). See the respective docstrings for details.
The main references for this are:
[L] Luke, Y. L. (1969), The Special Functions and Their Approximations,
Volume 1
[R] Kelly B. Roach. Meijer G Function Representations.
In: Proceedings of the 1997 International Symposium on Symbolic and
Algebraic Computation, pages 205-211, New York, 1997. ACM.
[P] A. P. Prudnikov, Yu. A. Brychkov and O. I. Marichev (1990).
Integrals and Series: More Special Functions, Vol. 3,.
Gordon and Breach Science Publisher
"""
from sympy.core import oo, S, pi
from sympy.core.function import expand, expand_mul, expand_power_base
from sympy.core.add import Add
from sympy.core.mul import Mul
from sympy.core.cache import cacheit
from sympy.core.symbol import Dummy, Wild
from sympy.simplify import hyperexpand, powdenest
from sympy.logic.boolalg import And, Or
from sympy.functions.special.delta_functions import Heaviside
from sympy.functions.elementary.piecewise import Piecewise
from sympy.functions.special.hyper import meijerg
from sympy.utilities.misc import debug as _debug
from sympy.utilities import default_sort_key
# keep this at top for easy reference
z = Dummy('z')
def _create_lookup_table(table):
""" Add formulae for the function -> meijerg lookup table. """
def wild(n):
return Wild(n, exclude=[z])
p, q, a, b, c = map(wild, 'pqabc')
n = Wild('n', properties=[lambda x: x.is_Integer and x > 0])
t = p*z**q
def add(formula, an, ap, bm, bq, arg=t, fac=S(1), cond=True, hint=True):
table.setdefault(_mytype(formula, z), []).append((formula,
[(fac, meijerg(an, ap, bm, bq, arg))], cond, hint))
def addi(formula, inst, cond, hint=True):
table.setdefault(_mytype(formula, z), []).append((formula, inst, cond, hint))
def constant(a):
return [(a, meijerg([1], [], [], [0], z)),
(a, meijerg([], [1], [0], [], z))]
table[()] = [(a, constant(a), True, True)]
# [P], Section 8.
from sympy import unpolarify, Function, Not
class IsNonPositiveInteger(Function):
nargs = 1
@classmethod
def eval(cls, arg):
arg = unpolarify(arg)
if arg.is_Integer is True:
return arg <= 0
# Section 8.4.2
from sympy import (gamma, pi, cos, exp, re, sin, sqrt, sinh, cosh,
factorial, log, erf, polar_lift)
# TODO this needs more polar_lift (c/f entry for exp)
add(Heaviside(t - b)*(t - b)**(a-1), [a], [], [], [0], t/b,
gamma(a)*b**(a-1), And(b > 0))
add(Heaviside(b - t)*(b - t)**(a-1), [], [a], [0], [], t/b,
gamma(a)*b**(a-1), And(b > 0))
add(Heaviside(z - (b/p)**(1/q))*(t - b)**(a-1), [a], [], [], [0], t/b,
gamma(a)*b**(a-1), And(b > 0))
add(Heaviside((b/p)**(1/q) - z)*(b - t)**(a-1), [], [a], [0], [], t/b,
gamma(a)*b**(a-1), And(b > 0))
add((b + t)**(-a), [1 - a], [], [0], [], t/b, b**(-a)/gamma(a),
hint=Not(IsNonPositiveInteger(a)))
add(abs(b - t)**(-a), [1 - a], [(1 - a)/2], [0], [(1 - a)/2], t/b,
pi/(gamma(a)*cos(pi*a/2))*abs(b)**(-a), re(a) < 1)
add((t**a - b**a)/(t - b), [0, a], [], [0, a], [], t/b,
b**(a-1)*sin(a*pi)/pi)
# 12
def A1(r, sign, nu): return pi**(-S(1)/2)*(-sign*nu/2)**(1-2*r)
def tmpadd(r, sgn):
# XXX the a**2 is bad for matching
add((sqrt(a**2 + t) + sgn*a)**b/(a**2+t)**r,
[(1 + b)/2, 1-2*r + b/2], [],
[(b - sgn*b)/2], [(b + sgn*b)/2], t/a**2,
a**(b-2*r)*A1(r, sgn, b))
tmpadd(0, 1)
tmpadd(0, -1)
tmpadd(S(1)/2, 1)
tmpadd(S(1)/2, -1)
# 13
def tmpadd(r, sgn):
add((sqrt(a + p*z**q) + sgn*sqrt(p)*z**(q/2))**b/(a + p*z**q)**r,
[1 - r + sgn*b/2], [1 - r - sgn*b/2], [0, S(1)/2], [],
p*z**q/a, a**(b/2 - r)*A1(r, sgn, b))
tmpadd(0, 1)
tmpadd(0, -1)
tmpadd(S(1)/2, 1)
tmpadd(S(1)/2, -1)
# (those after look obscure)
# Section 8.4.3
add(exp(polar_lift(-1)*t), [], [], [0], [])
# TODO can do sin^n, sinh^n by expansion ... where?
# 8.4.4 (hyperbolic functions)
add(sinh(t), [], [1], [S(1)/2], [1, 0], t**2/4, pi**(S(3)/2))
add(cosh(t), [], [S(1)/2], [0], [S(1)/2, S(1)/2], t**2/4, pi**(S(3)/2))
# Section 8.4.5
# TODO can do t + a. but can also do by expansion... (XXX not really)
add(sin(t), [], [], [S(1)/2], [0], t**2/4, sqrt(pi))
add(cos(t), [], [], [0], [S(1)/2], t**2/4, sqrt(pi))
# Section 8.5.5
def make_log1(subs):
N = subs[n]
return [((-1)**N*factorial(N),
meijerg([], [1]*(N + 1), [0]*(N + 1), [], t))]
def make_log2(subs):
N = subs[n]
return [(factorial(N),
meijerg([1]*(N + 1), [], [], [0]*(N + 1), t))]
# TODO these only hold for positive p, and can be made more general
# but who uses log(x)*Heaviside(a-x) anyway ...
# TODO also it would be nice to derive them recursively ...
addi(log(t)**n*Heaviside(1 - t), make_log1, True)
addi(log(t)**n*Heaviside(t - 1), make_log2, True)
def make_log3(subs):
return make_log1(subs) + make_log2(subs)
addi(log(t)**n, make_log3, True)
addi(log(t + a),
constant(log(a)) + [(S(1), meijerg([1, 1], [], [1], [0], t/a))],
True)
addi(log(abs(t - a)), constant(log(abs(a))) + \
[(pi, meijerg([1, 1], [S(1)/2], [1], [0, S(1)/2], t/a))],
True)
# TODO log(x)/(x+a) and log(x)/(x-1) can also be done. should they
# be derivable?
# TODO further formulae in this section seem obscure
# Sections 8.4.9-10
# TODO
# Section 8.4.11
from sympy import Ei, I, expint, Si, Ci, Shi, Chi, fresnels, fresnelc
addi(Ei(t),
constant(-I*pi) + [(S(-1), meijerg([], [1], [0, 0], [], t*polar_lift(-1)))],
True)
# Section 8.4.12
add(Si(t), [1], [], [S(1)/2], [0, 0], t**2/4, sqrt(pi)/2)
add(Ci(t), [], [1], [0, 0], [S(1)/2], t**2/4, -sqrt(pi)/2)
# Section 8.4.13
add(Shi(t), [S(1)/2], [], [0], [S(-1)/2, S(-1)/2], polar_lift(-1)*t**2/4,
t*sqrt(pi)/4)
add(Chi(t), [], [S(1)/2, 1], [0, 0], [S(1)/2, S(1)/2], t**2/4, -pi**S('3/2')/2)
# generalized exponential integral
add(expint(a, t), [], [a], [a - 1, 0], [], t)
# Section 8.4.14
# TODO erfc
add(erf(t), [1], [], [S(1)/2], [0], t**2, 1/sqrt(pi))
# TODO exp(-x)*erf(I*x) does not work
# Fresnel Integrals
add(fresnels(t), [1], [], [S(3)/4], [0, S(1)/4], pi**2*t**4/16, S(1)/2)
add(fresnelc(t), [1], [], [S(1)/4], [0, S(3)/4], pi**2*t**4/16, S(1)/2)
##### bessel-type functions #####
from sympy import besselj, bessely, besseli, besselk
# Section 8.4.19
add(besselj(a, t), [], [], [a/2], [-a/2], t**2/4)
# all of the following are derivable
#add(sin(t)*besselj(a, t), [S(1)/4, S(3)/4], [], [(1+a)/2],
# [-a/2, a/2, (1-a)/2], t**2, 1/sqrt(2))
#add(cos(t)*besselj(a, t), [S(1)/4, S(3)/4], [], [a/2],
# [-a/2, (1+a)/2, (1-a)/2], t**2, 1/sqrt(2))
#add(besselj(a, t)**2, [S(1)/2], [], [a], [-a, 0], t**2, 1/sqrt(pi))
#add(besselj(a, t)*besselj(b, t), [0, S(1)/2], [], [(a + b)/2],
# [-(a+b)/2, (a - b)/2, (b - a)/2], t**2, 1/sqrt(pi))
# Section 8.4.20
add(bessely(a, t), [], [-(a+1)/2], [a/2, -a/2], [-(a+1)/2], t**2/4)
# TODO all of the following should be derivable
#add(sin(t)*bessely(a, t), [S(1)/4, S(3)/4], [(1 - a - 1)/2],
# [(1 + a)/2, (1 - a)/2], [(1 - a - 1)/2, (1 - 1 - a)/2, (1 - 1 + a)/2],
# t**2, 1/sqrt(2))
#add(cos(t)*bessely(a, t), [S(1)/4, S(3)/4], [(0 - a - 1)/2],
# [(0 + a)/2, (0 - a)/2], [(0 - a - 1)/2, (1 - 0 - a)/2, (1 - 0 + a)/2],
# t**2, 1/sqrt(2))
#add(besselj(a, t)*bessely(b, t), [0, S(1)/2], [(a - b - 1)/2],
# [(a + b)/2, (a - b)/2], [(a - b - 1)/2, -(a + b)/2, (b - a)/2],
# t**2, 1/sqrt(pi))
#addi(bessely(a, t)**2,
# [(2/sqrt(pi), meijerg([], [S(1)/2, S(1)/2 - a], [0, a, -a],
# [S(1)/2 - a], t**2)),
# (1/sqrt(pi), meijerg([S(1)/2], [], [a], [-a, 0], t**2))],
# True)
#addi(bessely(a, t)*bessely(b, t),
# [(2/sqrt(pi), meijerg([], [0, S(1)/2, (1 - a - b)/2],
# [(a + b)/2, (a - b)/2, (b - a)/2, -(a + b)/2],
# [(1 - a - b)/2], t**2)),
# (1/sqrt(pi), meijerg([0, S(1)/2], [], [(a + b)/2],
# [-(a + b)/2, (a - b)/2, (b - a)/2], t**2))],
# True)
# Section 8.4.21 ?
# Section 8.4.22
add(besseli(a, t), [], [(1 + a)/2], [a/2], [-a/2, (1 + a)/2], t**2/4, pi)
# TODO many more formulas. should all be derivable
# Section 8.4.23
add(besselk(a, t), [], [], [a/2, -a/2], [], t**2/4, S(1)/2)
# TODO many more formulas. should all be derivable
####################################################################
# First some helper functions.
####################################################################
from sympy.utilities.timeutils import timethis
timeit = timethis('meijerg')
def _mytype(f, x):
""" Create a hashable entity describing the type of f. """
if not f.has(x):
return ()
elif f.is_Function:
return (type(f),)
else:
types = [_mytype(a, x) for a in f.args]
res = []
for t in types:
res += list(t)
res.sort()
return tuple(res)
class _CoeffExpValueError(ValueError):
"""
Exception raised by _get_coeff_exp, for internal use only.
"""
pass
def _get_coeff_exp(expr, x):
"""
When expr is known to be of the form c*x**b, with c and/or b possibly 1,
return c, b.
>>> from sympy.abc import x, a, b
>>> from sympy.integrals.meijerint import _get_coeff_exp
>>> _get_coeff_exp(a*x**b, x)
(a, b)
>>> _get_coeff_exp(x, x)
(1, 1)
>>> _get_coeff_exp(2*x, x)
(2, 1)
>>> _get_coeff_exp(x**3, x)
(1, 3)
"""
from sympy import powsimp
(c, m) = expand_power_base(powsimp(expr)).as_coeff_mul(x)
if not m:
return c, S(0)
[m] = m
if m.is_Pow:
if m.base != x:
raise _CoeffExpValueError('expr not of form a*x**b')
return c, m.exp
elif m == x:
return c, S(1)
else:
raise _CoeffExpValueError('expr not of form a*x**b: %s' % expr)
def _exponents(expr, x):
"""
Find the exponents of ``x`` (not including zero) in ``expr``.
>>> from sympy.integrals.meijerint import _exponents
>>> from sympy.abc import x, y
>>> from sympy import sin
>>> _exponents(x, x)
set([1])
>>> _exponents(x**2, x)
set([2])
>>> _exponents(x**2 + x, x)
set([1, 2])
>>> _exponents(x**3*sin(x + x**y) + 1/x, x)
set([-1, 1, 3, y])
"""
def _exponents_(expr, x, res):
if expr == x:
res.update([1])
return
if expr.is_Pow and expr.base == x:
res.update([expr.exp])
return
for arg in expr.args:
_exponents_(arg, x, res)
res = set()
_exponents_(expr, x, res)
return res
def _functions(expr, x):
""" Find the types of functions in expr, to estimate the complexity. """
from sympy import Function
return set(e.func for e in expr.atoms(Function) if e.has(x))
def _find_splitting_points(expr, x):
"""
Find numbers a such that a linear substitution x --> x+a would
(hopefully) simplify expr.
>>> from sympy.integrals.meijerint import _find_splitting_points as fsp
>>> from sympy import sin
>>> from sympy.abc import a, x
>>> fsp(x, x)
set([0])
>>> fsp((x-1)**3, x)
set([1])
>>> fsp(sin(x+3)*x, x)
set([-3, 0])
"""
from sympy import Tuple
p, q = map(lambda n: Wild(n, exclude=[x]), 'pq')
def compute_innermost(expr, res):
if isinstance(expr, Tuple):
return
m = expr.match(p*x+q)
if m and m[p] != 0:
res.add(-m[q]/m[p])
return
if expr.is_Atom:
return
for arg in expr.args:
compute_innermost(arg, res)
innermost = set()
compute_innermost(expr, innermost)
return innermost
def _split_mul(f, x):
"""
Split expression ``f`` into fac, po, g, where fac is a constant factor,
po = x**s for some s independent of s, and g is "the rest".
>>> from sympy.integrals.meijerint import _split_mul
>>> from sympy import sin
>>> from sympy.abc import s, x
>>> _split_mul((3*x)**s*sin(x**2)*x, x)
(3**s, x*x**s, sin(x**2))
"""
from sympy import polarify, unpolarify
fac = S(1)
po = S(1)
g = S(1)
f = expand_power_base(f)
args = Mul.make_args(f)
for a in args:
if a == x:
po *= x
elif not a.has(x):
fac *= a
else:
if a.is_Pow:
c, t = a.base.as_coeff_mul(x)
if t != (x,):
c, t = expand_mul(a.base).as_coeff_mul(x)
if t == (x,):
po *= x**a.exp
fac *= unpolarify(polarify(c**a.exp, subs=False))
continue
g *= a
return fac, po, g
def _mul_args(f):
"""
Return a list ``L`` such that Mul(*L) == f.
If f is not a Mul or Pow, L=[f].
If f=g**n for an integer n, L=[g]*n.
If f is a Mul, L comes from applying _mul_args to all factors of f.
"""
args = Mul.make_args(f)
gs = []
for g in args:
if g.is_Pow and g.exp.is_Integer:
n = g.exp
base = g.base
if n < 0:
n = -n
base = 1/base
gs += [base]*n
else:
gs.append(g)
return gs
def _mul_as_two_parts(f):
"""
Find all the ways to split f into a product of two terms.
Return None on failure.
>>> from sympy.integrals.meijerint import _mul_as_two_parts
>>> from sympy import sin, exp
>>> from sympy.abc import x
>>> _mul_as_two_parts(x*sin(x)*exp(x))
[(x*exp(x), sin(x)), (x, exp(x)*sin(x)), (x*sin(x), exp(x))]
"""
from sympy.utilities.iterables import multiset_partitions
gs = _mul_args(f)
if len(gs) < 2:
return None
return [(Mul(*x), Mul(*y)) for (x, y) in multiset_partitions(gs, 2)]
def _inflate_g(g, n):
""" Return C, h such that h is a G function of argument z**n and
g = C*h. """
# TODO should this be a method of meijerg?
# See: [L, page 150, equation (5)]
def inflate(params, n):
""" (a1, .., ak) -> (a1/n, (a1+1)/n, ..., (ak + n-1)/n) """
res = []
for a in params:
for i in range(n):
res.append((a + i)/n)
return res
v = S(len(g.ap) - len(g.bq))
C = n**(1 + g.nu + v/2)
C /= (2*pi)**((n - 1)*g.delta)
return C, meijerg(inflate(g.an, n), inflate(g.aother, n),
inflate(g.bm, n), inflate(g.bother, n),
g.argument**n * n**(n*v))
def _flip_g(g):
""" Turn the G function into one of inverse argument
(i.e. G(1/x) -> G'(x)) """
# See [L], section 5.2
def tr(l): return [1 - a for a in l]
return meijerg(tr(g.bm), tr(g.bother), tr(g.an), tr(g.aother), 1/g.argument)
def _inflate_fox_h(g, a):
r"""
Let d denote the integrand in the definition of the G function ``g``.
Consider the function H which is defined in the same way, but with
integrand d/Gamma(a*s) (contour conventions as usual).
If a is rational, the function H can be written as C*G, for a constant C
and a G-function G.
This function returns C, G.
"""
if a < 0:
return _inflate_fox_h(_flip_g(g), -a)
p = S(a.p)
q = S(a.q)
# We use the substitution s->qs, i.e. inflate g by q. We are left with an
# extra factor of Gamma(p*s), for which we use Gauss' multiplication
# theorem.
D, g = _inflate_g(g, q)
z = g.argument
D /= (2*pi)**((1-p)/2)*p**(-S(1)/2)
z /= p**p
bs = [(n+1)/p for n in range(p)]
return D, meijerg(g.an, g.aother, g.bm, list(g.bother) + bs, z)
_dummies = {}
def _dummy(name, token, expr, **kwargs):
"""
Return a dummy. This will return the same dummy if the same token+name is
requested more than once, and it is not already in expr.
This is for being cache-friendly.
"""
d = _dummy_(name, token, **kwargs)
if expr.has(d):
return Dummy(name, **kwargs)
return d
def _dummy_(name, token, **kwargs):
"""
Return a dummy associated to name and token. Same effect as declaring
it globally.
"""
global _dummies
if not (name, token) in _dummies:
_dummies[(name, token)] = Dummy(name, **kwargs)
return _dummies[(name, token)]
def _is_analytic(f, x):
""" Check if f(x), when expressed using G functions on the positive reals,
will in fact agree with the G functions almost everywhere """
from sympy import Heaviside, Abs
return not any(expr.has(x) for expr in f.atoms(Heaviside, Abs))
def _condsimp(cond):
"""
Do naive simplifications on ``cond``.
Note that this routine is completely ad-hoc, simplification rules being
added as need arises rather than following any logical pattern.
>>> from sympy.integrals.meijerint import _condsimp as simp
>>> from sympy import Or, Eq, unbranched_argument as arg, And
>>> from sympy.abc import x, y, z
>>> simp(Or(x < y, z, Eq(x, y)))
Or(x <= y, z)
>>> simp(Or(x <= y, And(x < y, z)))
x <= y
"""
from sympy import (symbols, Wild, Eq, unbranched_argument, exp_polar, pi, I,
periodic_argument, oo, polar_lift)
from sympy.logic.boolalg import BooleanFunction
if not isinstance(cond, BooleanFunction):
return cond
cond = cond.func(*map(_condsimp, cond.args))
change = True
p, q, r = symbols('p q r', cls=Wild)
rules = [
(Or(p < q, Eq(p, q)), p <= q),
# The next two obviously are instances of a general pattern, but it is
# easier to spell out the few cases we care about.
(And(abs(unbranched_argument(p)) <= pi,
abs(unbranched_argument(exp_polar(-2*pi*I)*p)) <= pi),
Eq(unbranched_argument(exp_polar(-I*pi)*p), 0)),
(And(abs(unbranched_argument(p)) <= pi/2,
abs(unbranched_argument(exp_polar(-pi*I)*p)) <= pi/2),
Eq(unbranched_argument(exp_polar(-I*pi/2)*p), 0)),
(Or(p <= q, And(p < q, r)), p <= q)
]
while change:
change = False
for fro, to in rules:
if fro.func != cond.func:
continue
for n, arg in enumerate(cond.args):
if fro.args[0].has(r):
m = arg.match(fro.args[1])
num = 1
else:
num = 0
m = arg.match(fro.args[0])
if not m:
continue
otherargs = map(lambda x: x.subs(m), fro.args[:num] + fro.args[num+1:])
otherlist = [n]
for arg2 in otherargs:
for k, arg3 in enumerate(cond.args):
if k in otherlist:
continue
if arg2 == arg3:
otherlist += [k]
break
if arg3.func is And and arg2.args[1] == r and \
arg2.func is And and arg2.args[0] in arg3.args:
otherlist += [k]
break
if arg3.func is And and arg2.args[0] == r and \
arg2.func is And and arg2.args[1] in arg3.args:
otherlist += [k]
break
if len(otherlist) != len(otherargs) + 1:
continue
newargs = [arg for (k, arg) in enumerate(cond.args) \
if k not in otherlist] + [to.subs(m)]
cond = cond.func(*newargs)
change = True
break
# final tweak
def repl_eq(orig):
if orig.lhs == 0:
expr = orig.rhs
elif orig.rhs == 0:
expr = orig.lhs
else:
return orig
m = expr.match(unbranched_argument(polar_lift(p)**q))
if not m:
if expr.func is periodic_argument and not expr.args[0].is_polar \
and expr.args[1] == oo:
return (expr.args[0] > 0)
return orig
return (m[p] > 0)
return cond.replace(lambda expr: expr.is_Relational and expr.rel_op == '==',
repl_eq)
def _eval_cond(cond):
""" Re-evaluate the conditions. """
if isinstance(cond, bool):
return cond
return _condsimp(cond.doit())
####################################################################
# Now the "backbone" functions to do actual integration.
####################################################################
def _my_principal_branch(expr, period, full_pb=False):
""" Bring expr nearer to its principal branch by removing superfluous
factors.
This function does *not* guarantee to yield the principal branch,
to avoid introducing opaque principal_branch() objects,
unless full_pb=True. """
from sympy import principal_branch
res = principal_branch(expr, period)
if not full_pb:
res = res.replace(principal_branch, lambda x, y: x)
return res
def _rewrite_saxena_1(fac, po, g, x):
"""
Rewrite the integral fac*po*g dx, from zero to infinity, as
integral fac*G, where G has argument a*x. Note po=x**s.
Return fac, G.
"""
_, s = _get_coeff_exp(po, x)
a, b = _get_coeff_exp(g.argument, x)
period = g.get_period()
a = _my_principal_branch(a, period)
# We substitute t = x**b.
C = fac/(abs(b)*a**((s+1)/b - 1))
# Absorb a factor of (at)**((1 + s)/b - 1).
def tr(l): return [a + (1 + s)/b - 1 for a in l]
return C, meijerg(tr(g.an), tr(g.aother), tr(g.bm), tr(g.bother),
a*x)
def _check_antecedents_1(g, x, helper=False):
"""
Return a condition under which the mellin transform of g exists.
Any power of x has already been absorbed into the G function,
so this is just int_0^\infty g dx.
See [L, section 5.6.1]. (Note that s=1.)
If ``helper`` is True, only check if the MT exists at infinity, i.e. if
int_1^\infty g dx exists.
"""
# NOTE if you update these conditions, please update the documentation as well
from sympy import Eq, Not, ceiling, Ne, re, unbranched_argument as arg
delta = g.delta
eta, _ = _get_coeff_exp(g.argument, x)
m, n, p, q = S([len(g.bm), len(g.an), len(g.ap), len(g.bq)])
xi = m + n - p
if p > q:
def tr(l): return [1 - x for x in l]
return _check_antecedents_1(meijerg(tr(g.bm), tr(g.bother),
tr(g.an), tr(g.aother), x/eta),
x)
tmp = []
for b in g.bm:
tmp += [-re(b) < 1]
for a in g.an:
tmp += [1 < 1 - re(a)]
cond_3 = And(*tmp)
for b in g.bother:
tmp += [-re(b) < 1]
for a in g.aother:
tmp += [1 < 1 - re(a)]
cond_3_star = And(*tmp)
cond_4 = (-re(g.nu) + (q + 1 - p)/2 > q - p)
def debug(*msg):
_debug(*msg)
debug('Checking antecedents for 1 function:')
debug(' delta=%s, eta=%s, m=%s, n=%s, p=%s, q=%s'
% (delta, eta, m, n, p, q))
debug(' ap = %s, %s' % (list(g.an), list(g.aother)))
debug(' bq = %s, %s' % (list(g.bm), list(g.bother)))
debug(' cond_3=%s, cond_3*=%s, cond_4=%s' % (cond_3, cond_3_star, cond_4))
conds = []
# case 1
case1 = []
tmp1 = [1 <= n, p < q, 1 <= m]
tmp2 = [1 <= p, 1 <= m, Eq(q, p + 1), Not(And(Eq(n, 0), Eq(m, p + 1)))]
tmp3 = [1 <= p, Eq(q, p)]
for k in range(ceiling(delta/2) + 1):
tmp3 += [Ne(abs(arg(eta)), (delta - 2*k)*pi)]
tmp = [delta > 0, abs(arg(eta)) < delta*pi]
extra = [Ne(eta, 0), cond_3]
if helper:
extra = []
for t in [tmp1, tmp2, tmp3]:
case1 += [And(*(t + tmp + extra))]
conds += case1
debug(' case 1:', case1)
# case 2
extra = [cond_3]
if helper:
extra = []
case2 = [And(Eq(n, 0), p + 1 <= m, m <= q,
abs(arg(eta)) < delta*pi, *extra)]
conds += case2
debug(' case 2:', case2)
# case 3
extra = [cond_3, cond_4]
if helper:
extra = []
case3 = [And(p < q, 1 <= m, delta > 0, Eq(abs(arg(eta)), delta*pi), *extra)]
case3 += [And(p <= q - 2, Eq(delta, 0), Eq(abs(arg(eta)), 0), *extra)]
conds += case3
debug(' case 3:', case3)
# TODO altered cases 4-7
# extra case from wofram functions site:
# (reproduced verbatim from prudnikov, section 2.24.2)
# http://functions.wolfram.com/HypergeometricFunctions/MeijerG/21/02/01/
case_extra = []
case_extra += [Eq(p, q), Eq(delta, 0), Eq(arg(eta), 0), Ne(eta, 0)]
if not helper:
case_extra += [cond_3]
s = []
for a, b in zip(g.ap, g.bq):
s += [b - a]
case_extra += [re(Add(*s)) < 0]
case_extra = And(*case_extra)
conds += [case_extra]
debug(' extra case:', [case_extra])
case_extra_2 = [And(delta > 0, abs(arg(eta)) < delta*pi)]
if not helper:
case_extra_2 += [cond_3]
case_extra_2 = And(*case_extra_2)
conds += [case_extra_2]
debug(' second extra case:', [case_extra_2])
# TODO This leaves only one case from the three listed by prudnikov.
# Investigate if these indeed cover everything; if so, remove the rest.
return Or(*conds)
def _int0oo_1(g, x):
"""
Evaluate int_0^\infty g dx using G functions,
assuming the necessary conditions are fulfilled.
>>> from sympy.abc import a, b, c, d, x, y
>>> from sympy import meijerg
>>> from sympy.integrals.meijerint import _int0oo_1
>>> _int0oo_1(meijerg([a], [b], [c], [d], x*y), x)
gamma(-a)*gamma(c + 1)/(y*gamma(-d)*gamma(b + 1))
"""
# See [L, section 5.6.1]. Note that s=1.
from sympy import gamma, combsimp, unpolarify
eta, _ = _get_coeff_exp(g.argument, x)
res = 1/eta
# XXX TODO we should reduce order first
for b in g.bm:
res *= gamma(b + 1)
for a in g.an:
res *= gamma(1 - a - 1)
for b in g.bother:
res /= gamma(1 - b - 1)
for a in g.aother:
res /= gamma(a + 1)
return combsimp(unpolarify(res))
def _rewrite_saxena(fac, po, g1, g2, x, full_pb=False):
"""
Rewrite the integral fac*po*g1*g2 from 0 to oo in terms of G functions
with argument c*x.
Return C, f1, f2 such that integral C f1 f2 from 0 to infinity equals
integral fac po g1 g2 from 0 to infinity.
>>> from sympy.integrals.meijerint import _rewrite_saxena
>>> from sympy.abc import s, t, m
>>> from sympy import meijerg
>>> g1 = meijerg([], [], [0], [], s*t)
>>> g2 = meijerg([], [], [m/2], [-m/2], t**2/4)
>>> r = _rewrite_saxena(1, t**0, g1, g2, t)
>>> r[0]
s/(4*sqrt(pi))
>>> r[1]
meijerg(((), ()), ((-1/2, 0), ()), s**2*t/4)
>>> r[2]
meijerg(((), ()), ((m/2,), (-m/2,)), t/4)
"""
from sympy.core.numbers import ilcm
def pb(g):
a, b = _get_coeff_exp(g.argument, x)
per = g.get_period()
return meijerg(g.an, g.aother, g.bm, g.bother,
_my_principal_branch(a, per, full_pb)*x**b)
_, s = _get_coeff_exp(po, x)
_, b1 = _get_coeff_exp(g1.argument, x)
_, b2 = _get_coeff_exp(g2.argument, x)
if b1 < 0:
b1 = -b1
g1 = _flip_g(g1)
if b2 < 0:
b2 = -b2
g2 = _flip_g(g2)
if not b1.is_Rational or not b2.is_Rational:
return
m1, n1 = b1.p, b1.q
m2, n2 = b2.p, b2.q
tau = ilcm(m1*n2, m2*n1)
r1 = tau//(m1*n2)
r2 = tau//(m2*n1)
C1, g1 = _inflate_g(g1, r1)
C2, g2 = _inflate_g(g2, r2)
g1 = pb(g1)
g2 = pb(g2)
fac *= C1*C2
a1, b = _get_coeff_exp(g1.argument, x)
a2, _ = _get_coeff_exp(g2.argument, x)
# arbitrarily tack on the x**s part to g1
# TODO should we try both?
exp = (s + 1)/b - 1
fac = fac/(abs(b) * a1**exp)
def tr(l): return [a + exp for a in l]
g1 = meijerg(tr(g1.an), tr(g1.aother), tr(g1.bm), tr(g1.bother), a1*x)
g2 = meijerg(g2.an, g2.aother, g2.bm, g2.bother, a2*x)
return powdenest(fac, polar=True), g1, g2
def _check_antecedents(g1, g2, x):
""" Return a condition under which the integral theorem applies. """
from sympy import (re, Eq, Not, Ne, cos, I, exp, ceiling, sin, sign,
unpolarify)
from sympy import arg as arg_, unbranched_argument as arg
# Yes, this is madness.
# XXX TODO this is a testing *nightmare*
# NOTE if you update these conditions, please update the documentation as well
# The following conditions are found in
# [P], Section 2.24.1
#
# They are also reproduced (verbatim!) at
# http://functions.wolfram.com/HypergeometricFunctions/MeijerG/21/02/03/
#
# Note: k=l=r=alpha=1
sigma, _ = _get_coeff_exp(g1.argument, x)
omega, _ = _get_coeff_exp(g2.argument, x)
s, t, u, v = S([len(g1.bm), len(g1.an), len(g1.ap), len(g1.bq)])
m, n, p, q = S([len(g2.bm), len(g2.an), len(g2.ap), len(g2.bq)])
bstar = s + t - (u + v)/2
cstar = m + n - (p + q)/2
rho = g1.nu + (u - v)/2 + 1
mu = g2.nu + (p - q)/2 + 1
phi = q - p - (v - u)
eta = 1 - (v - u) - mu - rho
psi = (pi*(q - m - n) + abs(arg(omega)))/(q - p)
theta = (pi*(v - s - t) + abs(arg(sigma)))/(v - u)
lambda_c = (q - p)*abs(omega)**(1/(q - p))*cos(psi) \
+ (v - u)*abs(sigma)**(1/(v - u))*cos(theta)
def lambda_s0(c1, c2):
return c1*(q-p)*abs(omega)**(1/(q-p))*sin(psi) \
+ c2*(v-u)*abs(sigma)**(1/(v-u))*sin(theta)
lambda_s = Piecewise(
((lambda_s0(+1, +1)*lambda_s0(-1, -1)),
And(Eq(arg(sigma), 0), Eq(arg(omega), 0))),
(lambda_s0(sign(arg(omega)), +1)*lambda_s0(sign(arg(omega)), -1),
And(Eq(arg(sigma), 0), Ne(arg(omega), 0))),
(lambda_s0(+1, sign(arg(sigma)))*lambda_s0(-1, sign(arg(sigma))),
And(Ne(arg(sigma), 0), Eq(arg(omega), 0))),
(lambda_s0(sign(arg(omega)), sign(arg(sigma))), True))
_debug('Checking antecedents:')
_debug(' sigma=%s, s=%s, t=%s, u=%s, v=%s, b*=%s, rho=%s'
% (sigma, s, t, u, v, bstar, rho))
_debug(' omega=%s, m=%s, n=%s, p=%s, q=%s, c*=%s, mu=%s,'
% (omega, m, n, p, q, cstar, mu))
_debug(' phi=%s, eta=%s, psi=%s, theta=%s' % (phi, eta, psi, theta))
c1 = True
for g in [g1, g2]:
for a in g1.an:
for b in g1.bm:
diff = a - b
if diff > 0 and diff.is_integer:
c1 = False
tmp = []
for b in g1.bm:
for d in g2.bm:
tmp += [re(1 + b + d) > 0]
c2 = And(*tmp)
tmp = []
for a in g1.an:
for c in g2.an:
tmp += [re(1 + a + c) < 1 + 1]
c3 = And(*tmp)
tmp = []
for c in g1.an:
tmp += [(p - q)*re(1 + c - 1) - re(mu) > -S(3)/2]
c4 = And(*tmp)
tmp = []
for d in g1.bm:
tmp += [(p - q)*re(1 + d) - re(mu) > -S(3)/2]
c5 = And(*tmp)
tmp = []
for c in g2.an:
tmp += [(u - v)*re(1 + c - 1) - re(rho) > -S(3)/2]
c6 = And(*tmp)
tmp = []
for d in g2.bm:
tmp += [(u - v)*re(1 + d) - re(rho) > -S(3)/2]
c7 = And(*tmp)
c8 = (abs(phi) + 2*re((rho - 1)*(q - p) + (v - u)*(q - p) + (mu - 1)*(v - u)) > 0)
c9 = (abs(phi) - 2*re((rho - 1)*(q - p) + (v - u)*(q - p) + (mu - 1)*(v - u)) > 0)
c10 = (abs(arg(sigma)) < bstar*pi)
c11 = Eq(abs(arg(sigma)), bstar*pi)
c12 = (abs(arg(omega)) < cstar*pi)
c13 = Eq(abs(arg(omega)), cstar*pi)
# The following condition is *not* implemented as stated on the wolfram
# function site. In the book of prudnikov there is an additional part
# (the And involving re()). However, I only have this book in russian, and
# I don't read any russian. The following condition is what other people
# have told me it means.
# Worryingly, it is different from the condition implemented in REDUCE.
# The REDUCE implementation:
# https://reduce-algebra.svn.sourceforge.net/svnroot/reduce-algebra/trunk/packages/defint/definta.red
# (search for tst14)
# The Wolfram alpha version:
# http://functions.wolfram.com/HypergeometricFunctions/MeijerG/21/02/03/03/0014/
z0 = exp(-(bstar + cstar)*pi*I)
zos = unpolarify(z0*omega/sigma)
zso = unpolarify(z0*sigma/omega)
if zos == 1/zso:
c14 = And(Eq(phi, 0), bstar + cstar <= 1,
Or(Ne(zos, 1), re(mu + rho + v - u) < 1,
re(mu + rho + q - p) < 1))
else:
c14 = And(Eq(phi, 0), bstar - 1 + cstar <= 0,
Or(And(Ne(zos, 1), abs(arg_(1 - zos)) < pi),
And(re(mu + rho + v - u) < 1, Eq(zos, 1))))
c14_alt = And(Eq(phi, 0), cstar - 1 + bstar <= 0,
Or(And(Ne(zso, 1), abs(arg_(1 - zso)) < pi),
And(re(mu + rho + q - p) < 1, Eq(zso, 1))))
# Since r=k=l=1, in our case there is c14_alt which is the same as calling
# us with (g1, g2) = (g2, g1). The conditions below enumerate all cases
# (i.e. we don't have to try arguments reversed by hand), and indeed try
# all symmetric cases. (i.e. whenever there is a condition involving c14,
# there is also a dual condition which is exactly what we would get when g1,
# g2 were interchanged, *but c14 was unaltered*).
# Hence the following seems correct:
c14 = Or(c14, c14_alt)
tmp = [lambda_c > 0,
And(Eq(lambda_c, 0), Ne(lambda_s, 0), re(eta) > -1),
And(Eq(lambda_c, 0), Eq(lambda_s, 0), re(eta) > 0)]
c15 = Or(*tmp)
if _eval_cond(lambda_c > 0) is not False:
c15 = (lambda_c > 0)
for cond, i in [(c1, 1), (c2, 2), (c3, 3), (c4, 4), (c5, 5), (c6, 6),
(c7, 7), (c8, 8), (c9, 9), (c10, 10), (c11, 11),
(c12, 12), (c13, 13), (c14, 14), (c15, 15)]:
_debug(' c%s:' % i, cond)
# We will return Or(*conds)
conds = []
def pr(count):
_debug(' case %s:' % count, conds[-1])
conds += [And(m*n*s*t != 0, bstar > 0, cstar > 0, c1, c2, c3, c10, c12)] #1
pr(1)
conds += [And(Eq(u, v), Eq(bstar, 0), cstar > 0, sigma > 0, re(rho) < 1,
c1, c2, c3, c12)] #2
pr(2)
conds += [And(Eq(p, q), Eq(cstar, 0), bstar > 0, omega > 0, re(mu) < 1,
c1, c2, c3, c10)] #3
pr(3)
conds += [And(Eq(p, q), Eq(u, v), Eq(bstar, 0), Eq(cstar, 0),
sigma > 0, omega > 0, re(mu) < 1, re(rho) < 1,
Ne(sigma, omega), c1, c2, c3)] #4
pr(4)
conds += [And(Eq(p, q), Eq(u, v), Eq(bstar, 0), Eq(cstar, 0),
sigma > 0, omega > 0, re(mu + rho) < 1,
Ne(omega, sigma), c1, c2, c3)] #5
pr(5)
conds += [And(p > q, s > 0, bstar > 0, cstar >= 0,
c1, c2, c3, c5, c10, c13)] #6
pr(6)
conds += [And(p < q, t > 0, bstar > 0, cstar >= 0,
c1, c2, c3, c4, c10, c13)] #7
pr(7)
conds += [And(u > v, m > 0, cstar > 0, bstar >= 0,
c1, c2, c3, c7, c11, c12)] #8
pr(8)
conds += [And(u < v, n > 0, cstar > 0, bstar >= 0,
c1, c2, c3, c6, c11, c12)] #9
pr(9)
conds += [And(p > q, Eq(u, v), Eq(bstar, 0), cstar >= 0, sigma > 0,
re(rho) < 1, c1, c2, c3, c5, c13)] #10
pr(10)
conds += [And(p < q, Eq(u, v), Eq(bstar, 0), cstar >= 0, sigma > 0,
re(rho) < 1, c1, c2, c3, c4, c13)] #11
pr(11)
conds += [And(Eq(p, q), u > v, bstar >= 0, Eq(cstar, 0), omega > 0,
re(mu) < 1, c1, c2, c3, c7, c11)] #12
pr(12)
conds += [And(Eq(p, q), u < v, bstar >= 0, Eq(cstar, 0), omega > 0,
re(mu) < 1, c1, c2, c3, c6, c11)] #13
pr(13)
conds += [And(p < q, u > v, bstar >= 0, cstar >= 0,
c1, c2,c3, c4, c7, c11, c13)] #14
pr(14)
conds += [And(p > q, u < v, bstar >= 0, cstar >= 0,
c1, c2, c3, c5, c6, c11, c13)] #15
pr(15)
conds += [And(p > q, u > v, bstar >= 0, cstar >= 0,
c1, c2, c3, c5, c7, c8, c11, c13, c14)] #16
pr(16)
conds += [And(p < q, u < v, bstar >= 0, cstar >= 0,
c1, c2, c3, c4, c6, c9, c11, c13, c14)] #17
pr(17)
conds += [And(Eq(t, 0), s > 0, bstar > 0, phi > 0, c1, c2, c10)] #18
pr(18)
conds += [And(Eq(s, 0), t > 0, bstar > 0, phi < 0, c1, c3, c10)] #19
pr(19)
conds += [And(Eq(n, 0), m > 0, cstar > 0, phi < 0, c1, c2, c12)] #20
pr(20)
conds += [And(Eq(m, 0), n > 0, cstar > 0, phi > 0, c1, c3, c12)] #21
pr(21)
conds += [And(Eq(s*t, 0), bstar > 0, cstar > 0,
c1, c2, c3, c10, c12)] #22
pr(22)
conds += [And(Eq(m*n, 0), bstar > 0, cstar > 0,
c1, c2, c3, c10, c12)] #23
pr(23)
# The following case is from [Luke1969]. As far as I can tell, it is *not*
# covered by prudnikov's.
# Let G1 and G2 be the two G-functions. Suppose the integral exists from
# 0 to a > 0 (this is easy the easy part), that G1 is exponential decay at
# infinity, and that the mellin transform of G2 exists.
# Then the integral exists.
mt1_exists = _check_antecedents_1(g1, x, helper=True)
mt2_exists = _check_antecedents_1(g2, x, helper=True)
conds += [And(mt2_exists, Eq(t, 0), u < s, bstar > 0, c10, c1, c2, c3)]
pr('E1')
conds += [And(mt2_exists, Eq(s, 0), v < t, bstar > 0, c10, c1, c2, c3)]
pr('E2')
conds += [And(mt1_exists, Eq(n, 0), p < m, cstar > 0, c12, c1, c2, c3)]
pr('E3')
conds += [And(mt1_exists, Eq(m, 0), q < n, cstar > 0, c12, c1, c2, c3)]
pr('E4')
# Let's short-circuit if this worked ...
# the rest is corner-cases and terrible to read.
r = Or(*conds)
if _eval_cond(r) is not False:
return r
conds += [And(m + n > p, Eq(t, 0), Eq(phi, 0), s > 0, bstar > 0, cstar < 0,
abs(arg(omega)) < (m + n - p + 1)*pi,
c1, c2, c10, c14, c15)] #24
pr(24)
conds += [And(m + n > q, Eq(s, 0), Eq(phi, 0), t > 0, bstar > 0, cstar < 0,
abs(arg(omega)) < (m + n - q + 1)*pi,
c1, c3, c10, c14, c15)] #25
pr(25)
conds += [And(Eq(p, q - 1), Eq(t, 0), Eq(phi, 0), s > 0, bstar > 0,
cstar >= 0, cstar*pi < abs(arg(omega)),
c1, c2, c10, c14, c15)] #26
pr(26)
conds += [And(Eq(p, q + 1), Eq(s, 0), Eq(phi, 0), t > 0, bstar > 0,
cstar >= 0, cstar*pi < abs(arg(omega)),
c1, c3, c10, c14, c15)] #27
pr(27)
conds += [And(p < q - 1, Eq(t, 0), Eq(phi, 0), s > 0, bstar > 0,
cstar >= 0, cstar*pi < abs(arg(omega)),
abs(arg(omega)) < (m + n - p + 1)*pi,
c1, c2, c10, c14, c15)] #28
pr(28)
conds += [And(p > q + 1, Eq(s, 0), Eq(phi, 0), t > 0, bstar > 0, cstar >= 0,
cstar*pi < abs(arg(omega)),
abs(arg(omega)) < (m + n - q + 1)*pi,
c1, c3, c10, c14, c15)] #29
pr(29)
conds += [And(Eq(n, 0), Eq(phi, 0), s + t > 0, m > 0, cstar > 0, bstar < 0,
abs(arg(sigma)) < (s + t - u + 1)*pi,
c1, c2, c12, c14, c15)] #30
pr(30)
conds += [And(Eq(m, 0), Eq(phi, 0), s + t > v, n > 0, cstar > 0, bstar < 0,
abs(arg(sigma)) < (s + t - v + 1)*pi,
c1, c3, c12, c14, c15)] #31
pr(31)
conds += [And(Eq(n, 0), Eq(phi, 0), Eq(u, v - 1), m > 0, cstar > 0,
bstar >= 0, bstar*pi < abs(arg(sigma)),
abs(arg(sigma)) < (bstar + 1)*pi,
c1, c2, c12, c14, c15)] #32
pr(32)
conds += [And(Eq(m, 0), Eq(phi, 0), Eq(u, v + 1), n > 0, cstar > 0,
bstar >= 0, bstar*pi < abs(arg(sigma)),
abs(arg(sigma)) < (bstar + 1)*pi,
c1, c3, c12, c14, c15)] #33
pr(33)
conds += [And(Eq(n, 0), Eq(phi, 0), u < v - 1, m > 0, cstar > 0, bstar >= 0,
bstar*pi < abs(arg(sigma)),
abs(arg(sigma)) < (s + t - u + 1)*pi,
c1, c2, c12, c14, c15)] #34
pr(34)
conds += [And(Eq(m, 0), Eq(phi, 0), u > v + 1, n > 0, cstar > 0, bstar >= 0,
bstar*pi < abs(arg(sigma)),
abs(arg(sigma)) < (s + t - v + 1)*pi,
c1, c3, c12, c14, c15)] #35
pr(35)
return Or(*conds)
# NOTE An alternative, but as far as I can tell weaker, set of conditions
# can be found in [L, section 5.6.2].
def _int0oo(g1, g2, x):
"""
Express integral from zero to infinity g1*g2 using a G function,
assuming the necessary conditions are fulfilled.
>>> from sympy.integrals.meijerint import _int0oo
>>> from sympy.abc import s, t, m
>>> from sympy import meijerg, S
>>> g1 = meijerg([], [], [-S(1)/2, 0], [], s**2*t/4)
>>> g2 = meijerg([], [], [m/2], [-m/2], t/4)
>>> _int0oo(g1, g2, t)
4*meijerg(((1/2, 0), ()), ((m/2,), (-m/2,)), s**(-2))/s**2
"""
# See: [L, section 5.6.2, equation (1)]
eta, _ = _get_coeff_exp(g1.argument, x)
omega, _ = _get_coeff_exp(g2.argument, x)
def neg(l): return [-x for x in l]
a1 = neg(g1.bm) + list(g2.an)
a2 = list(g2.aother) + neg(g1.bother)
b1 = neg(g1.an) + list(g2.bm)
b2 = list(g2.bother) + neg(g1.aother)
return meijerg(a1, a2, b1, b2, omega/eta)/eta
def _rewrite_inversion(fac, po, g, x):
""" Absorb ``po`` == x**s into g. """
_, s = _get_coeff_exp(po, x)
a, b = _get_coeff_exp(g.argument, x)
def tr(l): return [t + s/b for t in l]
return (powdenest(fac/a**(s/b), polar=True),
meijerg(tr(g.an), tr(g.aother), tr(g.bm), tr(g.bother), g.argument))
def _check_antecedents_inversion(g, x):
""" Check antecedents for the laplace inversion integral. """
from sympy import re, im, Or, And, Eq, exp, I, Add, nan, Ne
_debug('Checking antecedents for inversion:')
z = g.argument
_, e = _get_coeff_exp(z, x)
if e < 0:
_debug(' Flipping G.')
# We want to assume that argument gets large as |x| -> oo
return _check_antecedents_inversion(_flip_g(g), x)
def statement_half(a, b, c, z, plus):
coeff, exponent = _get_coeff_exp(z, x)
a *= exponent
b *= coeff**c
c *= exponent
conds = []
wp = b*exp(I*re(c)*pi/2)
wm = b*exp(-I*re(c)*pi/2)
if plus:
w = wp
else:
w = wm
conds += [And(Or(Eq(b, 0), re(c) <= 0), re(a) <= -1)]
conds += [And(Ne(b, 0), Eq(im(c), 0), re(c) > 0, re(w) < 0)]
conds += [And(Ne(b, 0), Eq(im(c), 0), re(c) > 0, re(w) <= 0,
re(a) <= -1)]
return Or(*conds)
def statement(a, b, c, z):
""" Provide a convergence statement for z**a * exp(b*z**c),
c/f sphinx docs. """
return And(statement_half(a, b, c, z, True),
statement_half(a, b, c, z, False))
# Notations from [L], section 5.7-10
m, n, p, q = S([len(g.bm), len(g.an), len(g.ap), len(g.bq)])
tau = m + n - p
nu = q - m - n
rho = (tau - nu)/2
sigma = q - p
if sigma == 1:
epsilon = S(1)/2
elif sigma > 1:
epsilon = 1
else:
epsilon = nan
theta = ((1 - sigma)/2 + Add(*g.bq) - Add(*g.ap))/sigma
delta = g.delta
_debug(' m=%s, n=%s, p=%s, q=%s, tau=%s, nu=%s, rho=%s, sigma=%s' % (
m, n, p, q, tau, nu, rho, sigma))
_debug(' epsilon=%s, theta=%s, delta=%s' % (epsilon, theta, delta))
# First check if the computation is valid.
if not (g.delta >= e/2 or (p >= 1 and p >= q)):
_debug(' Computation not valid for these parameters.')
return False
# Now check if the inversion integral exists.
# Test "condition A"
for a in g.an:
for b in g.bm:
if (a - b).is_integer and a > b:
_debug(' Not a valid G function.')
return False
# There are two cases. If p >= q, we can directly use a slater expansion
# like [L], 5.2 (11). Note in particular that the asymptotics of such an
# expansion even hold when some of the parameters differ by integers, i.e.
# the formula itself would not be valid! (b/c G functions are cts. in their
# parameters)
# When p < q, we need to use the theorems of [L], 5.10.
if p >= q:
_debug(' Using asymptotic slater expansion.')
return And(*[statement(a - 1, 0, 0, z) for a in g.an])
def E(z): return And(*[statement(a - 1, 0, z) for a in g.an])
def H(z): return statement(theta, -sigma, 1/sigma, z)
def Hp(z): return statement_half(theta, -sigma, 1/sigma, z, True)
def Hm(z): return statement_half(theta, -sigma, 1/sigma, z, False)
# [L], section 5.10
conds = []
# Theorem 1
conds += [And(1 <= n, p < q, 1 <= m, rho*pi - delta >= pi/2, delta > 0,
E(z*exp(I*pi*(nu + 1))))]
# Theorem 2, statements (2) and (3)
conds += [And(p + 1 <= m, m + 1 <= q, delta > 0, delta < pi/2, n == 0,
(m - p + 1)*pi - delta >= pi/2,
Hp(z*exp(I*pi*(q-m))), Hm(z*exp(-I*pi*(q-m))))]
# Theorem 2, statement (5)
conds += [And(p < q, m == q, n == 0, delta > 0,
(sigma + epsilon)*pi - delta >= pi/2, H(z))]
# Theorem 3, statements (6) and (7)
conds += [And(Or(And(p <= q - 2, 1 <= tau, tau <= sigma/2),
And(p + 1 <= m + n, m + n <= (p + q)/2)),
delta > 0, delta < pi/2, (tau + 1)*pi - delta >= pi/2,
Hp(z*exp(I*pi*nu)), Hm(z*exp(-I*pi*nu)))]
# Theorem 4, statements (10) and (11)
conds += [And(p < q, 1 <= m, rho > 0, delta > 0, delta + rho*pi < pi/2,
(tau + epsilon)*pi - delta >= pi/2,
Hp(z*exp(I*pi*nu)), Hm(z*exp(-I*pi*nu)))]
# Trivial case
conds += [m == 0]
# TODO
# Theorem 5 is quite general
# Theorem 6 contains special cases for q=p+1
return Or(*conds)
def _int_inversion(g, x, t):
"""
Compute the laplace inversion integral, assuming the formula applies.
"""
b, a = _get_coeff_exp(g.argument, x)
C, g = _inflate_fox_h(meijerg(g.an, g.aother, g.bm, g.bother, b/t**a), -a)
return C/t*g
####################################################################
# Finally, the real meat.
####################################################################
_lookup_table = None
@cacheit
@timeit
def _rewrite_single(f, x, recursive=True):
"""
Try to rewrite f as a sum of single G functions of the form
C*x**s*G(a*x**b), where b is a rational number and C is independent of x.
We guarantee that result.argument.as_coeff_mul(x) returns (a, (x**b,))
or (a, ()).
Returns a list of tuples (C, s, G) and a condition cond.
Returns None on failure.
"""
from sympy import polarify, unpolarify, oo, zoo, Tuple
global _lookup_table
if not _lookup_table:
_lookup_table = {}
_create_lookup_table(_lookup_table)
if isinstance(f, meijerg):
from sympy import factor
coeff, m = factor(f.argument, x).as_coeff_mul(x)
if len(m) > 1:
return None
m = m[0]
if m.is_Pow:
if m.base != x or not m.exp.is_Rational:
return None
elif m != x:
return None
return [(1, 0, meijerg(f.an, f.aother, f.bm, f.bother, coeff*m))], True
f_ = f
f = f.subs(x, z)
t = _mytype(f, z)
if t in _lookup_table:
l = _lookup_table[t]
for formula, terms, cond, hint in l:
subs = f.match(formula)
if subs:
subs_ = {}
for fro, to in subs.items():
subs_[fro] = unpolarify(polarify(to, lift=True),
exponents_only=True)
subs = subs_
if not isinstance(hint, bool):
hint = hint.subs(subs)
if hint is False:
continue
if not isinstance(cond, bool):
cond = unpolarify(cond.subs(subs))
if _eval_cond(cond) is False:
continue
if not isinstance(terms, list):
terms = terms(subs)
res = []
for fac, g in terms:
r1 = _get_coeff_exp(unpolarify(fac.subs(subs).subs(z, x),
exponents_only=True), x)
g = g.subs(subs).subs(z, x)
# NOTE these substitutions can in principle introduce oo,
# zoo and other absurdities. It shouldn't matter,
# but better be safe.
if Tuple(*(r1 + (g,))).has(oo, zoo, -oo):
continue
g = meijerg(g.an, g.aother, g.bm, g.bother,
unpolarify(g.argument, exponents_only=True))
res.append(r1 + (g,))
if res:
return res, cond
# try recursive mellin transform
if not recursive:
return None
_debug('Trying recursive mellin transform method.')
from sympy.integrals.transforms import (mellin_transform,
inverse_mellin_transform, IntegralTransformError,
MellinTransformStripError)
from sympy import oo, nan, zoo, simplify, cancel
def my_imt(F, s, x, strip):
""" Calling simplify() all the time is slow and not helpful, since
most of the time it only factors things in a way that has to be
un-done anyway. But sometimes it can remove apparent poles. """
# XXX should this be in inverse_mellin_transform?
try:
return inverse_mellin_transform(F, s, x, strip,
as_meijerg=True, needeval=True)
except MellinTransformStripError:
return inverse_mellin_transform(simplify(cancel(expand(F))), s, x, strip,
as_meijerg=True, needeval=True)
f = f_
s = _dummy('s', 'rewrite-single', f)
# to avoid infinite recursion, we have to force the two g functions case
def my_integrator(f, x):
from sympy import Integral, hyperexpand
r = _meijerint_definite_4(f, x, only_double=True)
if r is not None:
res, cond = r
res = _my_unpolarify(hyperexpand(res, rewrite='nonrepsmall'))
return Piecewise((res, cond),
(Integral(f, (x, 0, oo)), True))
return Integral(f, (x, 0, oo))
try:
F, strip, _ = mellin_transform(f, x, s, integrator=my_integrator,
simplify=False, needeval=True)
g = my_imt(F, s, x, strip)
except IntegralTransformError:
g = None
if g is None:
# We try to find an expression by analytic continuation.
# (also if the dummy is already in the expression, there is no point in
# putting in another one)
a = _dummy_('a', 'rewrite-single')
if not f.has(a) and _is_analytic(f, x):
try:
F, strip, _ = mellin_transform(f.subs(x, a*x), x, s,
integrator=my_integrator,
needeval=True, simplify=False)
g = my_imt(F, s, x, strip).subs(a, 1)
except IntegralTransformError:
g = None
if g is None or g.has(oo, nan, zoo):
_debug('Recursive mellin transform failed.')
return None
args = Add.make_args(g)
res = []
for f in args:
c, m = f.as_coeff_mul(x)
if len(m) > 1:
raise NotImplementedError('Unexpected form...')
g = m[0]
a, b = _get_coeff_exp(g.argument, x)
res += [(c, 0, meijerg(g.an, g.aother, g.bm, g.bother,
unpolarify(polarify(a, lift=True), exponents_only=True) \
*x**b))]
_debug('Recursive mellin transform worked:', g)
return res, True
def _rewrite1(f, x, recursive=True):
"""
Try to rewrite f using a (sum of) single G functions with argument a*x**b.
Return fac, po, g such that f = fac*po*g, fac is independent of x
and po = x**s.
Here g is a result from _rewrite_single.
Return None on failure.
"""
fac, po, g = _split_mul(f, x)
g = _rewrite_single(g, x, recursive)
if g:
return fac, po, g[0], g[1]
def _rewrite2(f, x):
"""
Try to rewrite f as a product of two G functions of arguments a*x**b.
Return fac, po, g1, g2 such that f = fac*po*g1*g2, where fac is
independent of x and po is x**s.
Here g1 and g2 are results of _rewrite_single.
Returns None on failure.
"""
fac, po, g = _split_mul(f, x)
if any(_rewrite_single(expr, x, False) is None for expr in _mul_args(g)):
return None
l = _mul_as_two_parts(g)
if not l:
return None
l.sort(key=lambda p: (max(len(_exponents(p[0], x)), len(_exponents(p[1], x))),
max(len(_functions(p[0], x)), len(_functions(p[1], x))),
max(len(_find_splitting_points(p[0], x)),
len(_find_splitting_points(p[1], x)))))
for recursive in [False, True]:
for fac1, fac2 in l:
g1 = _rewrite_single(fac1, x, recursive)
g2 = _rewrite_single(fac2, x, recursive)
if g1 and g2:
cond = And(g1[1], g2[1])
if cond is not False:
return fac, po, g1[0], g2[0], cond
def meijerint_indefinite(f, x):
"""
Compute an indefinite integral of ``f`` by rewriting it as a G function.
>>> from sympy.integrals.meijerint import meijerint_indefinite
>>> from sympy import sin
>>> from sympy.abc import x
>>> meijerint_indefinite(sin(x), x)
-cos(x)
"""
from sympy import hyper, meijerg, count_ops
results = []
for a in list(_find_splitting_points(f, x)) + [S(0)]:
res = _meijerint_indefinite_1(f.subs(x, x + a), x)
if res is None:
continue
results.append(res.subs(x, x - a))
if not res.has(hyper, meijerg):
return results[-1]
if results:
return sorted(results, key=count_ops)[0]
def _meijerint_indefinite_1(f, x):
""" Helper that does not attempt any substitution. """
from sympy import Integral, piecewise_fold
_debug('Trying to compute the indefinite integral of', f, 'wrt', x)
gs = _rewrite1(f, x)
if gs is None:
# Note: the code that calls us will do expand() and try again
return None
fac, po, gl, cond = gs
_debug(' could rewrite:', gs)
res = S(0)
for C, s, g in gl:
a, b = _get_coeff_exp(g.argument, x)
_, c = _get_coeff_exp(po, x)
c += s
# we do a substitution t=a*x**b, get integrand fac*t**rho*g
fac_ = fac * C / (b*a**((1 + c)/b))
rho = (c + 1)/b - 1
# we now use t**rho*G(params, t) = G(params + rho, t)
# [L, page 150, equation (4)]
# and integral G(params, t) dt = G(1, params+1, 0, t)
# (or a similar expression with 1 and 0 exchanged ... pick the one
# which yields a well-defined function)
# [R, section 5]
# (Note that this dummy will immediately go away again, so we
# can safely pass S(1) for ``expr``.)
t = _dummy('t', 'meijerint-indefinite', S(1))
def tr(p): return [a + rho + 1 for a in p]
if any(b.is_integer and b <= 0 for b in tr(g.bm)):
r = -meijerg(tr(g.an), tr(g.aother) + [1], tr(g.bm) + [0], tr(g.bother), t)
else:
r = meijerg(tr(g.an) + [1], tr(g.aother), tr(g.bm), tr(g.bother) + [0], t)
r = hyperexpand(r.subs(t, a*x**b))
# now substitute back
# Note: we really do want the powers of x to combine.
res += powdenest(fac_*r, polar=True)
def _clean(res):
"""This multiplies out superfluous powers of x we created, and chops off
constants:
>> _clean(x*(exp(x)/x - 1/x) + 3)
exp(x)
cancel is used before mul_expand since it is possible for an
expression to have an additive constant that doesn't become isolated
with simple expansion. Such a situation was identified in issue 3270:
>>> from sympy import sqrt, cancel
>>> from sympy.abc import x
>>> a = sqrt(2*x + 1)
>>> bad = (3*x*a**5 + 2*x - a**5 + 1)/a**2
>>> bad.expand().as_independent(x)[0]
0
>>> cancel(bad).expand().as_independent(x)[0]
1
"""
from sympy import cancel
res= expand_mul(cancel(res), deep=False)
return Add._from_args(res.as_coeff_add(x)[1])
res = piecewise_fold(res)
if res.is_Piecewise:
nargs = []
for expr, cond in res.args:
expr = _my_unpolarify(_clean(expr))
nargs += [(expr, cond)]
res = Piecewise(*nargs)
else:
res = _my_unpolarify(_clean(res))
return Piecewise((res, _my_unpolarify(cond)), (Integral(f, x), True))
@timeit
def meijerint_definite(f, x, a, b):
"""
Integrate ``f`` over the interval [``a``, ``b``], by rewriting it as a product
of two G functions, or as a single G function.
Return res, cond, where cond are convergence conditions.
>>> from sympy.integrals.meijerint import meijerint_definite
>>> from sympy import exp, oo
>>> from sympy.abc import x
>>> meijerint_definite(exp(-x**2), x, -oo, oo)
(sqrt(pi), True)
This function is implemented as a succession of functions
meijerint_definite, _meijerint_definite_2, _meijerint_definite_3,
_meijerint_definite_4. Each function in the list calls the next one
(presumably) several times. This means that calling meijerint_definite
can be very costly.
"""
# This consists of three steps:
# 1) Change the integration limits to 0, oo
# 2) Rewrite in terms of G functions
# 3) Evaluate the integral
#
# There are usually several ways of doing this, and we want to try all.
# This function does (1), calls _meijerint_definite_2 for step (2).
from sympy import Integral, arg, exp, I, And, DiracDelta, count_ops
_debug('Integrating', f, 'wrt %s from %s to %s.' % (x, a, b))
if f.has(DiracDelta):
_debug('Integrand has DiracDelta terms - giving up.')
return None
f_, x_, a_, b_ = f, x, a, b
# Let's use a dummy in case any of the boundaries has x.
d = Dummy('x')
f = f.subs(x, d)
x = d
if a == -oo and b != oo:
return meijerint_definite(f.subs(x, -x), x, -b, -a)
if a == -oo:
# Integrating -oo to oo. We need to find a place to split the integral.
_debug(' Integrating -oo to +oo.')
innermost = _find_splitting_points(f, x)
_debug(' Sensible splitting points:', innermost)
for c in sorted(innermost, key=default_sort_key, reverse=True) + [S(0)]:
_debug(' Trying to split at', c)
if not c.is_real:
_debug(' Non-real splitting point.')
continue
res1 = _meijerint_definite_2(f.subs(x, x + c), x)
if res1 is None:
_debug(' But could not compute first integral.')
continue
res2 = _meijerint_definite_2(f.subs(x, c-x), x)
if res2 is None:
_debug(' But could not compute second integral.')
continue
res1, cond1 = res1
res2, cond2 = res2
cond = _condsimp(And(cond1, cond2))
if cond is False:
_debug(' But combined condition is always false.')
continue
res = res1 + res2
return res, cond
return
if a == oo:
return -meijerint_definite(f, x, b, oo)
if (a, b) == (0, oo):
# This is a common case - try it directly first.
res = _meijerint_definite_2(f, x)
if res is not None and not res[0].has(meijerg):
return res
results = []
if b == oo:
for split in _find_splitting_points(f, x):
if (a - split >= 0) is True:
_debug('Trying x --> x + %s' % split)
res = _meijerint_definite_2(f.subs(x, x + split) \
*Heaviside(x + split - a), x)
if res is not None:
if res[0].has(meijerg):
results.append(res)
else:
return res
f = f.subs(x, x + a)
b = b - a
a = 0
if b != oo:
phi = exp(I*arg(b))
b = abs(b)
f = f.subs(x, phi*x)
f *= Heaviside(b - x)*phi
b = oo
_debug('Changed limits to', a, b)
_debug('Changed function to', f)
res = _meijerint_definite_2(f, x)
if res is not None:
if res[0].has(meijerg):
results.append(res)
else:
return res
if results:
return sorted(results, key=lambda x: count_ops(x[0]))[0]
def _guess_expansion(f, x):
""" Try to guess sensible rewritings for integrand f(x). """
from sympy import expand_trig
from sympy.functions.elementary.trigonometric import TrigonometricFunction
from sympy.functions.elementary.hyperbolic import HyperbolicFunction
res = [(f, 'originial integrand')]
expanded = expand_mul(res[-1][0])
if expanded != res[-1][0]:
res += [(expanded, 'expand_mul')]
expanded = expand(res[-1][0])
if expanded != res[-1][0]:
res += [(expanded, 'expand')]
if res[-1][0].has(TrigonometricFunction, HyperbolicFunction):
expanded = expand_mul(expand_trig(res[-1][0]))
if expanded != res[-1][0]:
res += [(expanded, 'expand_trig, expand_mul')]
return res
def _meijerint_definite_2(f, x):
"""
Try to integrate f dx from zero to infinty.
The body of this function computes various 'simplifications'
f1, f2, ... of f (e.g. by calling expand_mul(), trigexpand()
- see _guess_expansion) and calls _meijerint_definite_3 with each of
these in succession.
If _meijerint_definite_3 succeedes with any of the simplified functions,
returns this result.
"""
# This function does preparation for (2), calls
# _meijerint_definite_3 for (2) and (3) combined.
# use a positive dummy - we integrate from 0 to oo
dummy = _dummy('x', 'meijerint-definite2', f, positive=True)
f = f.subs(x, dummy)
x = dummy
if f == 0:
return S(0), True
for g, explanation in _guess_expansion(f, x):
_debug('Trying', explanation)
res = _meijerint_definite_3(g, x)
if res is not None and res[1] is not False:
return res
def _meijerint_definite_3(f, x):
"""
Try to integrate f dx from zero to infinity.
This function calls _meijerint_definite_4 to try to compute the
integral. If this fails, it tries using linearity.
"""
res = _meijerint_definite_4(f, x)
if res is not None and res[1] is not False:
return res
if f.is_Add:
_debug('Expanding and evaluating all terms.')
ress = [_meijerint_definite_4(g, x) for g in f.args]
if all(r is not None for r in ress):
conds = []
res = S(0)
for r, c in ress:
res += r
conds += [c]
c = And(*conds)
if c is not False:
return res, c
def _my_unpolarify(f):
from sympy import unpolarify
return _eval_cond(unpolarify(f))
@timeit
def _meijerint_definite_4(f, x, only_double=False):
"""
Try to integrate f dx from zero to infinity.
This function tries to apply the integration theorems found in literature,
i.e. it tries to rewrite f as either one or a product of two G-functions.
The parameter ``only_double`` is used internally in the recursive algorithm
to disable trying to rewrite f as a single G-function.
"""
# This function does (2) and (3)
_debug('Integrating', f)
# Try single G function.
if not only_double:
gs = _rewrite1(f, x, recursive=False)
if gs is not None:
fac, po, g, cond = gs
_debug('Could rewrite as single G function:', fac, po, g)
res = S(0)
for C, s, f in g:
if C == 0:
continue
C, f = _rewrite_saxena_1(fac*C, po*x**s, f, x)
res += C*_int0oo_1(f, x)
cond = And(cond, _check_antecedents_1(f, x))
cond = _my_unpolarify(cond)
_debug('Result before branch substitutions is:', res)
if cond is False:
_debug('But cond is always False.')
else:
return _my_unpolarify(hyperexpand(res)), cond
# Try two G functions.
gs = _rewrite2(f, x)
if gs is not None:
for full_pb in [False, True]:
fac, po, g1, g2, cond = gs
_debug('Could rewrite as two G functions:', fac, po, g1, g2)
res = S(0)
for C1, s1, f1 in g1:
for C2, s2, f2 in g2:
r = _rewrite_saxena(fac*C1*C2, po*x**(s1 + s2),
f1, f2, x, full_pb)
if r is None:
_debug('Non-rational exponents.')
return
C, f1_, f2_ = r
_debug('Saxena subst for yielded:', C, f1_, f2_)
cond = And(cond, _check_antecedents(f1_, f2_, x))
res += C*_int0oo(f1_, f2_, x)
_debug('Result before branch substitutions is:', res)
cond = _my_unpolarify(cond)
if cond is False:
_debug('But cond is always False (full_pb=%s).' % full_pb)
else:
if only_double:
return res, cond
return _my_unpolarify(hyperexpand(res)), cond
def meijerint_inversion(f, x, t):
"""
Compute the inverse laplace transform
:math:\int_{c+i\infty}^{c-i\infty} f(x) e^{tx) dx,
for real c larger than the real part of all singularities of f.
Note that ``t`` is always assumed real and positive.
Return None if the integral does not exist or could not be evaluated.
>>> from sympy.abc import x, t
>>> from sympy.integrals.meijerint import meijerint_inversion
>>> meijerint_inversion(1/x, x, t)
Heaviside(t)
"""
from sympy import I, Integral, exp, expand, log, Add, Mul, Heaviside
f_ = f
t_ = t
t = Dummy('t', polar=True) # We don't want sqrt(t**2) = abs(t) etc
f = f.subs(t_, t)
c = Dummy('c')
_debug('Laplace-inverting', f)
if not _is_analytic(f, x):
_debug('But expression is not analytic.')
return None
# We filter out exponentials here. If we are given an Add this will not
# work, but the calling code will take care of that.
shift = 0
if f.is_Mul:
args = list(f.args)
newargs = []
exponentials = []
while args:
arg = args.pop()
if isinstance(arg, exp):
arg2 = expand(arg)
if arg2.is_Mul:
args += arg2.args
continue
try:
a, b = _get_coeff_exp(arg.args[0], x)
except _CoeffExpValueError:
b = 0
if b == 1:
exponentials.append(a)
else:
newargs.append(arg)
elif arg.is_Pow:
arg2 = expand(arg)
if arg2.is_Mul:
args += arg2.args
continue
if not arg.base.has(x):
try:
a, b = _get_coeff_exp(arg.exp, x)
except _CoeffExpValueError:
b = 0
if b == 1:
exponentials.append(a*log(arg.base))
newargs.append(arg)
else:
newargs.append(arg)
shift = Add(*exponentials)
f = Mul(*newargs)
gs = _rewrite1(f, x)
if gs is not None:
fac, po, g, cond = gs
_debug('Could rewrite as single G function:', fac, po, g)
res = S(0)
for C, s, f in g:
C, f = _rewrite_inversion(fac*C, po*x**s, f, x)
res += C*_int_inversion(f, x, t)
cond = And(cond, _check_antecedents_inversion(f, x))
cond = _my_unpolarify(cond)
if cond is False:
_debug('But cond is always False.')
else:
_debug('Result before branch substitution:', res)
res = _my_unpolarify(hyperexpand(res))
if not res.has(Heaviside):
res *= Heaviside(t)
res = res.subs(t, t + shift)
if not isinstance(cond, bool):
cond = cond.subs(t, t + shift)
return Piecewise((res.subs(t, t_), cond),
(Integral(f_*exp(x*t), (x, c - oo*I, c + oo*I)).subs(t, t_), True))
| flacjacket/sympy | sympy/integrals/meijerint.py | Python | bsd-3-clause | 70,440 |
"""Contains implementations for each ported operation in Pandas.
Attributes:
decoder_ (NumPyEncoder): Description
encoder_ (NumPyDecoder): Description
"""
from encoders import *
from weld.weldobject import *
encoder_ = NumPyEncoder()
decoder_ = NumPyDecoder()
def get_field(expr, field):
""" Fetch a field from a struct expr
"""
weld_obj = WeldObject(encoder_, decoder_)
struct_var = weld_obj.update(expr)
if isinstance(expr, WeldObject):
struct_var = expr.obj_id
weld_obj.dependencies[struct_var] = expr
weld_template = """
%(struct)s.$%(field)s
"""
weld_obj.weld_code = weld_template % {"struct":struct_var,
"field":field}
return weld_obj
def unique(array, ty):
"""
Returns a new array-of-arrays with all duplicate arrays removed.
Args:
array (WeldObject / Numpy.ndarray): Input array
ty (WeldType): Type of each element in the input array
Returns:
A WeldObject representing this computation
"""
weld_obj = WeldObject(encoder_, decoder_)
array_var = weld_obj.update(array)
if isinstance(array, WeldObject):
array_var = array.obj_id
weld_obj.dependencies[array_var] = array
weld_template = """
map(
tovec(
result(
for(
map(
%(array)s,
|p: %(ty)s| {p,0}
),
dictmerger[%(ty)s,i32,+],
|b, i, e| merge(b,e)
)
)
),
|p: {%(ty)s, i32}| p.$0
)
"""
weld_obj.weld_code = weld_template % {"array": array_var, "ty": ty}
return weld_obj
def aggr(array, op, initial_value, ty):
"""
Returns sum of elements in the array.
Args:
array (WeldObject / Numpy.ndarray): Input array
op (str): Op string used to aggregate the array (+ / *)
initial_value (int): Initial value for aggregation
ty (WeldType): Type of each element in the input array
Returns:
A WeldObject representing this computation
"""
weld_obj = WeldObject(encoder_, decoder_)
array_var = weld_obj.update(array)
if isinstance(array, WeldObject):
array_var = array.obj_id
weld_obj.dependencies[array_var] = array
weld_template = """
result(
for(
%(array)s,
merger[%(ty)s,%(op)s],
|b, i, e| merge(b, e)
)
)
"""
weld_obj.weld_code = weld_template % {
"array": array_var, "ty": ty, "op": op}
return weld_obj
def mask(array, predicates, new_value, ty):
"""
Returns a new array, with each element in the original array satisfying the
passed-in predicate set to `new_value`
Args:
array (WeldObject / Numpy.ndarray): Input array
predicates (WeldObject / Numpy.ndarray<bool>): Predicate set
new_value (WeldObject / Numpy.ndarray / str): mask value
ty (WeldType): Type of each element in the input array
Returns:
A WeldObject representing this computation
"""
weld_obj = WeldObject(encoder_, decoder_)
array_var = weld_obj.update(array)
if isinstance(array, WeldObject):
array_var = array.obj_id
weld_obj.dependencies[array_var] = array
predicates_var = weld_obj.update(predicates)
if isinstance(predicates, WeldObject):
predicates_var = predicates.obj_id
weld_obj.dependencies[predicates_var] = predicates
if str(ty).startswith("vec"):
new_value_var = weld_obj.update(new_value)
if isinstance(new_value, WeldObject):
new_value_var = new_value.obj_id
weld_obj.dependencies[new_value_var] = new_value
else:
new_value_var = "%s(%s)" % (ty, str(new_value))
weld_template = """
map(
zip(%(array)s, %(predicates)s),
|p: {%(ty)s, bool}| if (p.$1, %(new_value)s, p.$0)
)
"""
weld_obj.weld_code = weld_template % {
"array": array_var,
"predicates": predicates_var,
"new_value": new_value_var,
"ty": ty}
return weld_obj
def filter(array, predicates, ty=None):
"""
Returns a new array, with each element in the original array satisfying the
passed-in predicate set to `new_value`
Args:
array (WeldObject / Numpy.ndarray): Input array
predicates (WeldObject / Numpy.ndarray<bool>): Predicate set
ty (WeldType): Type of each element in the input array
Returns:
A WeldObject representing this computation
"""
weld_obj = WeldObject(encoder_, decoder_)
array_var = weld_obj.update(array)
if isinstance(array, WeldObject):
array_var = array.obj_id
weld_obj.dependencies[array_var] = array
predicates_var = weld_obj.update(predicates)
if isinstance(predicates, WeldObject):
predicates_var = predicates.obj_id
weld_obj.dependencies[predicates_var] = predicates
weld_template = """
result(
for(
zip(%(array)s, %(predicates)s),
appender,
|b, i, e| if (e.$1, merge(b, e.$0), b)
)
)
"""
weld_obj.weld_code = weld_template % {
"array": array_var,
"predicates": predicates_var}
return weld_obj
def pivot_filter(pivot_array, predicates, ty=None):
"""
Returns a new array, with each element in the original array satisfying the
passed-in predicate set to `new_value`
Args:
array (WeldObject / Numpy.ndarray): Input array
predicates (WeldObject / Numpy.ndarray<bool>): Predicate set
ty (WeldType): Type of each element in the input array
Returns:
A WeldObject representing this computation
"""
weld_obj = WeldObject(encoder_, decoder_)
pivot_array_var = weld_obj.update(pivot_array)
if isinstance(pivot_array, WeldObject):
pivot_array_var = pivot_array.obj_id
weld_obj.dependencies[pivot_array_var] = pivot_array
predicates_var = weld_obj.update(predicates)
if isinstance(predicates, WeldObject):
predicates_var = predicates.obj_id
weld_obj.dependencies[predicates_var] = predicates
weld_template = """
let index_filtered =
result(
for(
zip(%(array)s.$0, %(predicates)s),
appender,
|b, i, e| if (e.$1, merge(b, e.$0), b)
)
);
let pivot_filtered =
map(
%(array)s.$1,
|x|
result(
for(
zip(x, %(predicates)s),
appender,
|b, i, e| if (e.$1, merge(b, e.$0), b)
)
)
);
{index_filtered, pivot_filtered, %(array)s.$2}
"""
weld_obj.weld_code = weld_template % {
"array": pivot_array_var,
"predicates": predicates_var}
return weld_obj
def isin(array, predicates, ty):
""" Checks if elements in array is also in predicates
# TODO: better parameter naming
Args:
array (WeldObject / Numpy.ndarray): Input array
predicates (WeldObject / Numpy.ndarray<bool>): Predicate set
ty (WeldType): Type of each element in the input array
Returns:
A WeldObject representing this computation
"""
weld_obj = WeldObject(encoder_, decoder_)
array_var = weld_obj.update(array)
if isinstance(array, WeldObject):
array_var = array.obj_id
weld_obj.dependencies[array_var] = array
predicates_var = weld_obj.update(predicates)
if isinstance(predicates, WeldObject):
predicates_var = predicates.obj_id
weld_obj.dependencies[predicates_var] = predicates
weld_template = """
let check_dict =
result(
for(
map(
%(predicate)s,
|p: %(ty)s| {p,0}
),
dictmerger[%(ty)s,i32,+],
|b, i, e| merge(b,e)
)
);
map(
%(array)s,
|x: %(ty)s| keyexists(check_dict, x)
)
"""
weld_obj.weld_code = weld_template % {
"array": array_var,
"predicate": predicates_var,
"ty": ty}
return weld_obj
def element_wise_op(array, other, op, ty):
"""
Operation of series and other, element-wise (binary operator add)
Args:
array (WeldObject / Numpy.ndarray): Input array
other (WeldObject / Numpy.ndarray): Second Input array
op (str): Op string used to compute element-wise operation (+ / *)
ty (WeldType): Type of each element in the input array
Returns:
A WeldObject representing this computation
"""
weld_obj = WeldObject(encoder_, decoder_)
array_var = weld_obj.update(array)
if isinstance(array, WeldObject):
array_var = array.obj_id
weld_obj.dependencies[array_var] = array
other_var = weld_obj.update(other)
if isinstance(other, WeldObject):
other_var = other.obj_id
weld_obj.dependencies[other_var] = other
weld_template = """
map(
zip(%(array)s, %(other)s),
|a| a.$0 %(op)s a.$1
)
"""
weld_obj.weld_code = weld_template % {"array": array_var,
"other": other_var,
"ty": ty, "op": op}
return weld_obj
def unzip_columns(expr, column_types):
"""
Zip together multiple columns.
Args:
columns (WeldObject / Numpy.ndarray): lust of columns
Returns:
A WeldObject representing this computation
"""
weld_obj = WeldObject(encoder_, decoder_)
column_appenders = []
struct_fields = []
result_fields = []
for i, column_type in enumerate(column_types):
column_appenders.append("appender[%s]" % column_type)
struct_fields.append("merge(b.$%s, e.$%s)" % (i, i))
result_fields.append("result(unzip_builder.$%s)" % i)
appender_string = "{%s}" % ", ".join(column_appenders)
struct_string = "{%s}" % ", ".join(struct_fields)
result_string = "{%s}" % ", ".join(result_fields)
expr_var = weld_obj.update(expr)
if isinstance(expr, WeldObject):
expr_var = expr.obj_id
weld_obj.dependencies[expr_var] = expr
weld_template = """
let unzip_builder = for(
%(expr)s,
%(appenders)s,
|b,i,e| %(struct_builder)s
);
%(result)s
"""
weld_obj.weld_code = weld_template % {"expr": expr_var,
"appenders": appender_string,
"struct_builder": struct_string,
"result": result_string}
return weld_obj
def sort(expr, field = None, keytype=None, ascending=True):
"""
Sorts the vector.
If the field parameter is provided then the sort
operators on a vector of structs where the sort key
is the field of the struct.
Args:
expr (WeldObject)
field (Int)
"""
weld_obj = WeldObject(encoder_, decoder_)
expr_var = weld_obj.update(expr)
if isinstance(expr, WeldObject):
expr_var = expr.obj_id
weld_obj.dependencies[expr_var] = expr
if field is not None:
key_str = "x.$%s" % field
else:
key_str = "x"
if not ascending:
# The type is not necessarily f64.
key_str = key_str + "* %s(-1)" % keytype
weld_template = """
sort(%(expr)s, |x| %(key)s)
"""
weld_obj.weld_code = weld_template % {"expr":expr_var, "key":key_str}
return weld_obj
def slice_vec(expr, start, stop):
"""
Slices the vector.
Args:
expr (WeldObject)
start (Long)
stop (Long)
"""
weld_obj = WeldObject(encoder_, decoder_)
expr_var = weld_obj.update(expr)
if isinstance(expr, WeldObject):
expr_var = expr.obj_id
weld_obj.dependencies[expr_var] = expr
weld_template = """
slice(%(expr)s, %(start)sL, %(stop)sL)
"""
weld_obj.weld_code = weld_template % {"expr":expr_var,
"start":start,
"stop":stop}
return weld_obj
def zip_columns(columns):
"""
Zip together multiple columns.
Args:
columns (WeldObject / Numpy.ndarray): lust of columns
Returns:
A WeldObject representing this computation
"""
weld_obj = WeldObject(encoder_, decoder_)
column_vars = []
for column in columns:
col_var = weld_obj.update(column)
if isinstance(column, WeldObject):
col_var = column.obj_id
weld_obj.dependencies[col_var] = column
column_vars.append(col_var)
arrays = ", ".join(column_vars)
weld_template = """
result(
for(
zip(%(array)s),
appender,
|b, i, e| merge(b, e)
)
)
"""
weld_obj.weld_code = weld_template % {
"array": arrays,
}
return weld_obj
def compare(array, other, op, ty_str):
"""
Performs passed-in comparison op between every element in the passed-in
array and other, and returns an array of booleans.
Args:
array (WeldObject / Numpy.ndarray): Input array
other (WeldObject / Numpy.ndarray): Second input array
op (str): Op string used for element-wise comparison (== >= <= !=)
ty (WeldType): Type of each element in the input array
Returns:
A WeldObject representing this computation
"""
weld_obj = WeldObject(encoder_, decoder_)
array_var = weld_obj.update(array)
if isinstance(array, WeldObject):
array_var = array.obj_id
weld_obj.dependencies[array_var] = array
# Strings need to be encoded into vec[char] array.
# Constants can be added directly to NVL snippet.
if isinstance(other, str) or isinstance(other, WeldObject):
other_var = weld_obj.update(other)
if isinstance(other, WeldObject):
other_var = tmp.obj_id
weld_obj.dependencies[other_var] = other
else:
other_var = "%s(%s)" % (ty_str, str(other))
weld_template = """
map(
%(array)s,
|a: %(ty)s| a %(op)s %(other)s
)
"""
weld_obj.weld_code = weld_template % {"array": array_var,
"other": other_var,
"op": op, "ty": ty_str}
return weld_obj
def slice(array, start, size, ty):
"""
Returns a new array-of-arrays with each array truncated, starting at
index `start` for `length` characters.
Args:
array (WeldObject / Numpy.ndarray): Input array
start (int): starting index
size (int): length to truncate at
ty (WeldType): Type of each element in the input array
Returns:
A WeldObject representing this computation
"""
weld_obj = WeldObject(encoder_, decoder_)
array_var = weld_obj.update(array)
if isinstance(array, WeldObject):
array_var = array.obj_id
weld_obj.dependencies[array_var] = array
weld_template = """
map(
%(array)s,
|array: %(ty)s| slice(array, %(start)dL, %(size)dL)
)
"""
weld_obj.weld_code = weld_template % {"array": array_var, "start": start,
"ty": ty, "size": size}
return weld_obj
def to_lower(array, ty):
"""
Returns a new array of strings that are converted to lowercase
Args:
array (WeldObject / Numpy.ndarray): Input array
start (int): starting index
size (int): length to truncate at
ty (WeldType): Type of each element in the input array
Returns:
A WeldObject representing this computation
"""
weld_obj = WeldObject(encoder_, decoder_)
array_var = weld_obj.update(array)
if isinstance(array, WeldObject):
array_var = array.obj_id
weld_obj.dependencies[array_var] = array
weld_template = """
map(
%(array)s,
|array: vec[i8]| map(array, |b:i8| if(b <= i8(90), b + i8(32), b))
)
"""
weld_obj.weld_code = weld_template % {"array": array_var, "ty": ty}
return weld_obj
def contains(array, ty, string):
"""
Checks if given string is contained in each string in the array.
Output is a vec of booleans.
Args:
array (WeldObject / Numpy.ndarray): Input array
start (int): starting index
size (int): length to truncate at
ty (WeldType): Type of each element in the input array
Returns:
A WeldObject representing this computation
"""
weld_obj = WeldObject(encoder_, decoder_)
string_obj = weld_obj.update(string)
if isinstance(string, WeldObject):
string_obj = string.obj_id
weld_obj.dependencies[string_obj] = string
array_var = weld_obj.update(array)
if isinstance(array, WeldObject):
array_var = array.obj_id
weld_obj.dependencies[array_var] = array
(start, end) = 0, len(string)
# Some odd bug where iterating on str and slicing str results
# in a segfault
weld_template = """
map(
%(array)s,
|str: vec[%(ty)s]|
let tstr = str;
result(
for(
str,
merger[i8,+](i8(0)),
|b,i,e|
if(slice(tstr, i, i64(%(end)s)) == %(cmpstr)s,
merge(b, i8(1)),
b
)
)
) > i8(0)
)
"""
weld_obj.weld_code = weld_template % {"array": array_var, "ty": ty,
"start": start, "end": end,
"cmpstr": string_obj}
return weld_obj
def count(array, ty):
"""
Return number of non-NA/null observations in the Series
TODO : Filter out NaN's once Weld supports NaN's
Args:
array (WeldObject / Numpy.ndarray): Input array
ty (WeldType): Type of each element in the input array
Returns:
A WeldObject representing this computation
"""
weld_obj = WeldObject(encoder_, decoder_)
array_var = weld_obj.update(array)
if isinstance(array, WeldObject):
array_var = array.obj_id
weld_obj.dependencies[array_var] = array
weld_template = """
len(%(array)s)
"""
weld_obj.weld_code = weld_template % {"array": array_var}
return weld_obj
def join(expr1, expr2, d1_keys, d2_keys, keys_type, d1_vals, df1_vals_ty, d2_vals, df2_vals_ty):
"""
Computes a join on two tables
"""
weld_obj = WeldObject(encoder_, decoder_)
df1_var = weld_obj.update(expr1)
if isinstance(expr1, WeldObject):
df1_var = expr1.obj_id
weld_obj.dependencies[df1_var] = expr1
df2_var = weld_obj.update(expr2)
if isinstance(expr2, WeldObject):
df2_var = expr2.obj_id
weld_obj.dependencies[df2_var] = expr2
d1_key_fields = ", ".join(["e.$%s" % k for k in d1_keys])
if len(d1_keys) > 1:
d1_key_struct = "{%s}" % (d1_key_fields)
else:
d1_key_struct = d1_key_fields
d1_val_fields = ", ".join(["e.$%s" % k for k in d1_vals])
d2_key_fields = ", ".join(["e.$%s" % k for k in d2_keys])
if len(d2_keys) > 1:
d2_key_struct = "{%s}" % (d2_key_fields)
else:
d2_key_struct = d2_key_fields
d2_val_fields = ", ".join(["e.$%s" % k for k in d2_vals])
d2_val_fields2 = ", ".join(["e2.$%s" % i for i, k in enumerate(d2_vals)])
d2_val_struct = "{%s}" % (d2_val_fields)
weld_template = """
let df2_join_table = result(
for(
%(df2)s,
groupmerger[%(kty)s, %(df2ty)s],
|b, i, e| merge(b, {%(df2key)s, %(df2vals)s})
)
);
result(for(
%(df1)s,
appender,
|b, i, e|
for(
lookup(df2_join_table, %(df1key)s),
b,
|b2, i2, e2| merge(b, {%(df1key)s, %(df1vals)s, %(df2vals2)s})
)
))
"""
weld_obj.weld_code = weld_template % {"df1":df1_var,
"df1key":d1_key_struct,
"df1vals": d1_val_fields,
"df2":df2_var,
"kty":keys_type,
"df2ty":df2_vals_ty,
"df2key":d2_key_struct,
"df2vals":d2_val_struct,
"df2vals2":d2_val_fields2}
return weld_obj
def pivot_table(expr, value_index, value_ty, index_index, index_ty, columns_index, columns_ty, aggfunc):
"""
Constructs a pivot table where the index_index and columns_index are used as keys and the value_index is used as the value which is aggregated.
"""
weld_obj = WeldObject(encoder_, decoder_)
zip_var = weld_obj.update(expr)
if isinstance(expr, WeldObject):
zip_var = expr.obj_id
weld_obj.dependencies[zip_var] = expr
if aggfunc == 'sum':
weld_template = """
let bs = for(
%(zip_expr)s,
{dictmerger[{%(ity)s,%(cty)s},%(vty)s,+], dictmerger[%(ity)s,i64,+], dictmerger[%(cty)s,i64,+]},
|b, i, e| {merge(b.$0, {{e.$%(id)s, e.$%(cd)s}, e.$%(vd)s}),
merge(b.$1, {e.$%(id)s, 1L}),
merge(b.$2, {e.$%(cd)s, 1L})}
);
let agg_dict = result(bs.$0);
let ind_vec = sort(map(tovec(result(bs.$1)), |x| x.$0), |x, y| compare(x,y));
let col_vec = map(tovec(result(bs.$2)), |x| x.$0);
let pivot = map(
col_vec,
|x:%(cty)s|
map(ind_vec, |y:%(ity)s| f64(lookup(agg_dict, {y, x})))
);
{ind_vec, pivot, col_vec}
"""
elif aggfunc == 'mean':
weld_template = """
let bs = for(
%(zip_expr)s,
{dictmerger[{%(ity)s,%(cty)s},{%(vty)s, i64},+], dictmerger[%(ity)s,i64,+], dictmerger[%(cty)s,i64,+]},
|b, i, e| {merge(b.$0, {{e.$%(id)s, e.$%(cd)s}, {e.$%(vd)s, 1L}}),
merge(b.$1, {e.$%(id)s, 1L}),
merge(b.$2, {e.$%(cd)s, 1L})}
);
let agg_dict = result(bs.$0);
let ind_vec = sort(map(tovec(result(bs.$1)), |x| x.$0), |x, y| compare(x,y));
let col_vec = map(tovec(result(bs.$2)), |x| x.$0);
let pivot = map(
col_vec,
|x:%(cty)s|
map(ind_vec, |y:%(ity)s| (
let sum_len_pair = lookup(agg_dict, {y, x});
f64(sum_len_pair.$0) / f64(sum_len_pair.$1))
)
);
{ind_vec, pivot, col_vec}
"""
else:
raise Exception("Aggregate operation %s not supported." % aggfunc)
weld_obj.weld_code = weld_template % {"zip_expr": zip_var,
"ity": index_ty,
"cty": columns_ty,
"vty": value_ty,
"id": index_index,
"cd": columns_index,
"vd": value_index}
return weld_obj
def get_pivot_column(pivot, column_name, column_type):
weld_obj = WeldObject(encoder_, decoder_)
pivot_var = weld_obj.update(pivot)
if isinstance(pivot, WeldObject):
pivot_var = pivot.obj_id
weld_obj.dependencies[pivot_var] = pivot
column_name_var = weld_obj.update(column_name)
if isinstance(column_name, WeldObject):
column_name_var = column_name.obj_id
weld_obj.dependencies[column_name_var] = column_name
weld_template = """
let col_dict = result(for(
%(piv)s.$2,
dictmerger[%(cty)s,i64,+],
|b, i, e| merge(b, {e, i})
));
{%(piv)s.$0, lookup(%(piv)s.$1, lookup(col_dict, %(colnm)s))}
"""
weld_obj.weld_code = weld_template % {"piv":pivot_var,
"cty":column_type,
"colnm":column_name_var}
return weld_obj
def pivot_sort(pivot, column_name, index_type, column_type, pivot_type):
weld_obj = WeldObject(encoder_, decoder_)
pivot_var = weld_obj.update(pivot)
if isinstance(pivot, WeldObject):
pivot_var = pivot.obj_id
weld_obj.dependencies[pivot_var] = pivot
column_name_var = weld_obj.update(column_name)
if isinstance(column_name, WeldObject):
column_name_var = column_name.obj_id
weld_obj.dependencies[column_name_var] = column_name
# Note the key_column is hardcoded for the movielens workload
# diff is located at the 2nd index.
weld_template = """
let key_col = lookup(%(piv)s.$1, 2L);
let sorted_indices = map(
sort(
result(
for(
key_col,
appender[{%(pvty)s,i64}],
|b,i,e| merge(b, {e,i})
)
),
|x,y| compare(x.$0, y.$0)
),
|x| x.$1
);
let new_piv = map(
%(piv)s.$1,
|x| result(
for(
x,
appender[%(pvty)s],
|b,i,e| merge(b, lookup(x, lookup(sorted_indices, i)))
)
)
);
let new_index = result(
for(
%(piv)s.$0,
appender[%(idty)s],
|b,i,e| merge(b, lookup(%(piv)s.$0, lookup(sorted_indices, i)))
)
);
{new_index, new_piv, %(piv)s.$2}
"""
weld_obj.weld_code = weld_template % {"piv": pivot_var,
"cty": column_type,
"idty": index_type,
"colnm": column_name_var,
"pvty": pivot_type}
return weld_obj
def set_pivot_column(pivot, column_name, item, pivot_type, column_type):
weld_obj = WeldObject(encoder_, decoder_)
pivot_var = weld_obj.update(pivot)
if isinstance(pivot, WeldObject):
pivot_var = pivot.obj_id
weld_obj.dependencies[pivot_var] = pivot
column_name_var = weld_obj.update(column_name)
if isinstance(column_name, WeldObject):
column_name_var = column_name.obj_id
weld_obj.dependencies[column_name_var] = column_name
item_var = weld_obj.update(item)
if isinstance(item, WeldObject):
item_var = item.obj_id
weld_obj.dependencies[item_var] = item
weld_template = """
let pv_cols = for(
%(piv)s.$1,
appender[%(pvty)s],
|b, i, e| merge(b, e)
);
let col_names = for(
%(piv)s.$2,
appender[%(cty)s],
|b, i, e| merge(b, e)
);
let new_pivot_cols = result(merge(pv_cols, %(item)s));
let new_col_names = result(merge(col_names, %(colnm)s));
{%(piv)s.$0, new_pivot_cols, new_col_names}
"""
weld_obj.weld_code = weld_template % {"piv":pivot_var,
"cty":column_type,
"pvty":pivot_type,
"item":item_var,
"colnm":column_name_var}
return weld_obj
def pivot_sum(pivot, value_type):
weld_obj = WeldObject(encoder_, decoder_)
pivot_var = weld_obj.update(pivot)
if isinstance(pivot, WeldObject):
pivot_var = pivot.obj_id
weld_obj.dependencies[pivot_var] = pivot
weld_template = """
result(for(
%(piv)s.$0,
appender[%(pty)s],
|b1,i1,e1|
merge(b1,
result(for(
%(piv)s.$1,
merger[%(pty)s,+],
|b2, i2, e2| merge(b2, lookup(e2, i1))
))
)
))
"""
weld_obj.weld_code = weld_template % {"piv":pivot_var,
"pty":value_type}
return weld_obj
def pivot_div(pivot, series, vec_type, val_type):
weld_obj = WeldObject(encoder_, decoder_)
pivot_var = weld_obj.update(pivot)
if isinstance(pivot, WeldObject):
pivot_var = pivot.obj_id
weld_obj.dependencies[pivot_var] = pivot
series_var = weld_obj.update(series)
if isinstance(series, WeldObject):
series_var = series.obj_id
weld_obj.dependencies[series_var] = series
weld_template = """
{%(piv)s.$0,
map(
%(piv)s.$1,
|v:%(vty)s|
result(for(
v,
appender[f64],
|b, i, e| merge(b, f64(e) / f64(lookup(%(srs)s, i)))
))
),
%(piv)s.$2
}
"""
weld_obj.weld_code = weld_template % {"piv":pivot_var,
"pty":val_type,
"vty":vec_type,
"srs":series_var}
return weld_obj
def groupby_sum(columns, column_tys, grouping_columns, grouping_column_tys):
"""
Groups the given columns by the corresponding grouping column
value, and aggregate by summing values.
Args:
columns (List<WeldObject>): List of columns as WeldObjects
column_tys (List<str>): List of each column data ty
grouping_column (WeldObject): Column to group rest of columns by
Returns:
A WeldObject representing this computation
"""
weld_obj = WeldObject(encoder_, decoder_)
if len(grouping_columns) == 1 and len(grouping_column_tys) == 1:
grouping_column_var = weld_obj.update(grouping_columns[0])
if isinstance(grouping_columns[0], WeldObject):
grouping_column_var = grouping_columns[0].weld_code
grouping_column_ty_str = "%s" % grouping_column_tys[0]
else:
grouping_column_vars = []
for column in grouping_columns:
column_var = weld_obj.update(column)
if isinstance(column_var, WeldObject):
column_var = column_var.weld_code
grouping_column_vars.append(column_var)
grouping_column_var = ", ".join(grouping_column_vars)
grouping_column_tys = [str(ty) for ty in grouping_column_tys]
grouping_column_ty_str = ", ".join(grouping_column_tys)
grouping_column_ty_str = "{%s}" % grouping_column_ty_str
columns_var_list = []
for column in columns:
column_var = weld_obj.update(column)
if isinstance(column, WeldObject):
column_var = column.obj_id
weld_obj.dependencies[column_var] = column
columns_var_list.append(column_var)
if len(columns_var_list) == 1 and len(grouping_columns) == 1:
columns_var = columns_var_list[0]
tys_str = column_tys[0]
result_str = "merge(b, e)"
elif len(columns_var_list) == 1 and len(grouping_columns) > 1:
columns_var = columns_var_list[0]
tys_str = column_tys[0]
key_str_list = []
for i in xrange(0, len(grouping_columns)):
key_str_list.append("e.$%d" % i)
key_str = "{%s}" % ", ".join(key_str_list)
value_str = "e.$" + str(len(grouping_columns))
result_str_list = [key_str, value_str]
result_str = "merge(b, {%s})" % ", ".join(result_str_list)
elif len(columns_var_list) > 1 and len(grouping_columns) == 1:
columns_var = "%s" % ", ".join(columns_var_list)
column_tys = [str(ty) for ty in column_tys]
tys_str = "{%s}" % ", ".join(column_tys)
key_str = "e.$0"
value_str_list = []
for i in xrange(1, len(columns) + 1):
value_str_list.append("e.$%d" % i)
value_str = "{%s}" % ", ".join(value_str_list)
result_str_list = [key_str, value_str]
result_str = "merge(b, {%s})" % ", ".join(result_str_list)
else:
columns_var = "%s" % ", ".join(columns_var_list)
column_tys = [str(ty) for ty in column_tys]
tys_str = "{%s}" % ", ".join(column_tys)
key_str_list = []
key_size = len(grouping_columns)
value_size = len(columns)
for i in xrange(0, key_size):
key_str_list.append("e.$%d" % i)
key_str = "{%s}" % ", ".join(key_str_list)
value_str_list = []
for i in xrange(key_size, key_size + value_size):
value_str_list.append("e.$%d" % i)
value_str = "{%s}" % ", ".join(value_str_list)
result_str_list = [key_str, value_str]
result_str = "merge(b, {%s})" % ", ".join(result_str_list)
weld_template = """
tovec(
result(
for(
zip(%(grouping_column)s, %(columns)s),
dictmerger[%(gty)s, %(ty)s, +],
|b, i, e| %(result)s
)
)
)
"""
weld_obj.weld_code = weld_template % {"grouping_column": grouping_column_var,
"columns": columns_var,
"result": result_str,
"ty": tys_str,
"gty": grouping_column_ty_str}
return weld_obj
def groupby_std(columns, column_tys, grouping_columns, grouping_column_tys):
"""
Groups the given columns by the corresponding grouping column
value, and aggregate by summing values.
Args:
columns (List<WeldObject>): List of columns as WeldObjects
column_tys (List<str>): List of each column data ty
grouping_column (WeldObject): Column to group rest of columns by
Returns:
A WeldObject representing this computation
"""
weld_obj = WeldObject(encoder_, decoder_)
if len(grouping_columns) == 1 and len(grouping_column_tys) == 1:
grouping_column_var = weld_obj.update(grouping_columns[0])
if isinstance(grouping_columns[0], WeldObject):
grouping_column_var = grouping_columns[0].weld_code
grouping_column_ty_str = "%s" % grouping_column_tys[0]
else:
grouping_column_vars = []
for column in grouping_columns:
column_var = weld_obj.update(column)
if isinstance(column_var, WeldObject):
column_var = column_var.weld_code
grouping_column_vars.append(column_var)
grouping_column_var = ", ".join(grouping_column_vars)
grouping_column_tys = [str(ty) for ty in grouping_column_tys]
grouping_column_ty_str = ", ".join(grouping_column_tys)
grouping_column_ty_str = "{%s}" % grouping_column_ty_str
columns_var_list = []
for column in columns:
column_var = weld_obj.update(column)
if isinstance(column, WeldObject):
column_var = column.obj_id
weld_obj.dependencies[column_var] = column
columns_var_list.append(column_var)
if len(columns_var_list) == 1 and len(grouping_columns) == 1:
columns_var = columns_var_list[0]
tys_str = column_tys[0]
result_str = "merge(b, e)"
# TODO : The above change needs to apply for all result strings
# These currently break at the moment
elif len(columns_var_list) == 1 and len(grouping_columns) > 1:
columns_var = columns_var_list[0]
tys_str = column_tys[0]
key_str_list = []
for i in xrange(0, len(grouping_columns)):
key_str_list.append("e.$%d" % i)
key_str = "{%s}" % ", ".join(key_str_list)
value_str = "e.$" + str(len(grouping_columns))
result_str_list = [key_str, value_str]
result_str = "merge(b, {%s, 1L})" % ", ".join(result_str_list)
elif len(columns_var_list) > 1 and len(grouping_columns) == 1:
columns_var = "%s" % ", ".join(columns_var_list)
column_tys = [str(ty) for ty in column_tys]
tys_str = "{%s}" % ", ".join(column_tys)
key_str = "e.$0"
value_str_list = []
for i in xrange(1, len(columns) + 1):
value_str_list.append("e.$%d" % i)
value_str = "{%s}" % ", ".join(value_str_list)
result_str_list = [key_str, value_str]
result_str = "merge(b, %s)" % ", ".join(result_str_list)
else:
columns_var = "%s" % ", ".join(columns_var_list)
column_tys = [str(ty) for ty in column_tys]
tys_str = "{%s}" % ", ".join(column_tys)
key_str_list = []
key_size = len(grouping_columns)
value_size = len(columns)
for i in xrange(0, key_size):
key_str_list.append("e.$%d" % i)
key_str = "{%s}" % ", ".join(key_str_list)
value_str_list = []
for i in xrange(key_size, key_size + value_size):
value_str_list.append("e.$%d" % i)
value_str = "{%s}" % ", ".join(value_str_list)
result_str_list = [key_str, value_str]
result_str = "merge(b, %s)" % ", ".join(result_str_list)
# TODO Need to implement exponent operator
# The mean dict's e.$1.$0 assumes this is a scalar vector and not a struct vector
# Also pandas normalizes by n - 1
weld_template = """
let sum_dict = result(
for(
zip(%(grouping_column)s, %(columns)s),
groupmerger[%(gty)s, %(ty)s],
|b, i, e| %(result)s
)
);
map(
sort(tovec(sum_dict), |x| x.$0),
|e| {e.$0,
(let sum = result(for(e.$1, merger[%(ty)s,+], |b,i,a| merge(b, a)));
let m = f64(sum) / f64(len(e.$1));
let msqr = result(for(e.$1, merger[f64,+], |b,i,a| merge(b, (f64(a)-m)*(f64(a)-m))));
sqrt(msqr / f64(len(e.$1) - 1L))
)}
)
"""
weld_obj.weld_code = weld_template % {"grouping_column": grouping_column_var,
"columns": columns_var,
"result": result_str,
"ty": tys_str,
"gty": grouping_column_ty_str}
return weld_obj
def groupby_size(columns, column_tys, grouping_columns, grouping_column_tys):
"""
Groups the given columns by the corresponding grouping column
value, and aggregate by summing values.
Args:
columns (List<WeldObject>): List of columns as WeldObjects
column_tys (List<str>): List of each column data ty
grouping_column (WeldObject): Column to group rest of columns by
Returns:
A WeldObject representing this computation
"""
weld_obj = WeldObject(encoder_, decoder_)
if len(grouping_columns) == 1 and len(grouping_column_tys) == 1:
grouping_column_var = weld_obj.update(grouping_columns[0])
if isinstance(grouping_columns[0], WeldObject):
grouping_column_var = grouping_columns[0].weld_code
grouping_column_ty_str = "%s" % grouping_column_tys[0]
else:
grouping_column_vars = []
for column in grouping_columns:
column_var = weld_obj.update(column)
if isinstance(column_var, WeldObject):
column_var = column_var.weld_code
grouping_column_vars.append(column_var)
grouping_column_var = ", ".join(grouping_column_vars)
grouping_column_var = "zip(%s)" % grouping_column_var
grouping_column_tys = [str(ty) for ty in grouping_column_tys]
grouping_column_ty_str = ", ".join(grouping_column_tys)
grouping_column_ty_str = "{%s}" % grouping_column_ty_str
weld_template = """
let group = sort(
tovec(
result(
for(
%(grouping_column)s,
dictmerger[%(gty)s, i64, +],
|b, i, e| merge(b, {e, 1L})
)
)
),
|x:{%(gty)s, i64}, y:{%(gty)s, i64}| compare(x.$0, y.$0)
);
{
map(
group,
|x:{%(gty)s,i64}| x.$0
),
map(
group,
|x:{%(gty)s,i64}| x.$1
)
}
"""
weld_obj.weld_code = weld_template % {"grouping_column": grouping_column_var,
"gty": grouping_column_ty_str}
return weld_obj
def groupby_sort(columns, column_tys, grouping_columns, grouping_column_tys, key_index, ascending):
"""
Groups the given columns by the corresponding grouping column
value, and aggregate by summing values.
Args:
columns (List<WeldObject>): List of columns as WeldObjects
column_tys (List<str>): List of each column data ty
grouping_column (WeldObject): Column to group rest of columns by
Returns:
A WeldObject representing this computation
"""
weld_obj = WeldObject(encoder_, decoder_)
if len(grouping_columns) == 1 and len(grouping_column_tys) == 1:
grouping_column_var = weld_obj.update(grouping_columns[0])
if isinstance(grouping_columns[0], WeldObject):
grouping_column_var = grouping_columns[0].weld_code
grouping_column_ty_str = "%s" % grouping_column_tys[0]
else:
grouping_column_vars = []
for column in grouping_columns:
column_var = weld_obj.update(column)
if isinstance(column, WeldObject):
column_var = column.obj_id
weld_obj.dependencies[column_var] = column
grouping_column_vars.append(column_var)
grouping_column_var = ", ".join(grouping_column_vars)
grouping_column_tys = [str(ty) for ty in grouping_column_tys]
grouping_column_ty_str = ", ".join(grouping_column_tys)
grouping_column_ty_str = "{%s}" % grouping_column_ty_str
columns_var_list = []
for column in columns:
column_var = weld_obj.update(column)
if isinstance(column, WeldObject):
column_var = column.obj_id
weld_obj.dependencies[column_var] = column
columns_var_list.append(column_var)
if len(columns_var_list) == 1 and len(grouping_columns) == 1:
columns_var = columns_var_list[0]
tys_str = column_tys[0]
result_str = "merge(b, e)"
elif len(columns_var_list) == 1 and len(grouping_columns) > 1:
columns_var = columns_var_list[0]
tys_str = column_tys[0]
key_str_list = []
for i in xrange(0, len(grouping_columns)):
key_str_list.append("e.$%d" % i)
key_str = "{%s}" % ", ".join(key_str_list)
value_str = "e.$" + str(len(grouping_columns))
result_str_list = [key_str, value_str]
result_str = "merge(b, {%s})" % ", ".join(result_str_list)
elif len(columns_var_list) > 1 and len(grouping_columns) == 1:
columns_var = "%s" % ", ".join(columns_var_list)
column_tys = [str(ty) for ty in column_tys]
tys_str = "{%s}" % ", ".join(column_tys)
key_str = "e.$0"
value_str_list = []
for i in xrange(1, len(columns) + 1):
value_str_list.append("e.$%d" % i)
value_str = "{%s}" % ", ".join(value_str_list)
result_str_list = [key_str, value_str]
result_str = "merge(b, {%s})" % ", ".join(result_str_list)
else:
columns_var = "%s" % ", ".join(columns_var_list)
column_tys = [str(ty) for ty in column_tys]
tys_str = "{%s}" % ", ".join(column_tys)
key_str_list = []
key_size = len(grouping_columns)
value_size = len(columns)
for i in xrange(0, key_size):
key_str_list.append("e.$%d" % i)
key_str = "{%s}" % ", ".join(key_str_list)
value_str_list = []
for i in xrange(key_size, key_size + value_size):
value_str_list.append("e.$%d" % i)
value_str = "{%s}" % ", ".join(value_str_list)
result_str_list = [key_str, value_str]
result_str = "merge(b, {%s})" % ", ".join(result_str_list)
if key_index == None:
key_str_x = "x"
key_str_y = "y"
else :
key_str_x = "x.$%d" % key_index
key_str_y = "y.$%d" % key_index
if ascending == False:
key_str = key_str + "* %s(-1)" % column_tys[key_index]
weld_template = """
@(grain_size:1)map(
tovec(
result(
for(
zip(%(grouping_column)s, %(columns)s),
groupmerger[%(gty)s, %(ty)s],
|b, i, e| %(result)s
)
)
),
|a:{%(gty)s, vec[%(ty)s]}| {a.$0, sort(a.$1, |x:%(ty)s, y:%(ty)s| compare(%(key_str_x)s, %(key_str_y)s))}
)
"""
weld_obj.weld_code = weld_template % {"grouping_column": grouping_column_var,
"columns": columns_var,
"result": result_str,
"ty": tys_str,
"gty": grouping_column_ty_str,
"key_str_x": key_str_x,
"key_str_y": key_str_y}
return weld_obj
def flatten_group(expr, column_tys, grouping_column_tys):
"""
Groups the given columns by the corresponding grouping column
value, and aggregate by summing values.
Args:
columns (List<WeldObject>): List of columns as WeldObjects
column_tys (List<str>): List of each column data ty
grouping_column (WeldObject): Column to group rest of columns by
Returns:
A WeldObject representing this computation
"""
weld_obj = WeldObject(encoder_, decoder_)
group_var = weld_obj.update(expr)
num_group_cols = len(grouping_column_tys)
if num_group_cols == 1:
grouping_column_ty_str = "%s" % grouping_column_tys[0]
grouping_column_key_str = "a.$0"
else:
grouping_column_tys = [str(ty) for ty in grouping_column_tys]
grouping_column_ty_str = ", ".join(grouping_column_tys)
grouping_column_ty_str = "{%s}" % grouping_column_ty_str
grouping_column_keys = ["a.$0.$%s" % i for i in range(0, num_group_cols)]
grouping_column_key_str = "{%s}" % ", ".join(grouping_column_keys)
if len(column_tys) == 1:
tys_str = "%s" % column_tys[0]
column_values_str = "b.$1"
else:
column_tys = [str(ty) for ty in column_tys]
tys_str = "{%s}" % ", ".join(column_tys)
column_values = ["b.$%s" % i for i in range(0, len(column_tys))]
column_values_str = "{%s}" % ", ".join(column_values)
# TODO: The output in pandas keps the keys sorted (even if keys are structs)
# We need to allow keyfunctions in sort by clauses to be able to compare structs.
# sort(%(group_vec)s, |x:{%(gty)s, vec[%(ty)s]}| x.$0),
weld_template = """
flatten(
map(
%(group_vec)s,
|a:{%(gty)s, vec[%(ty)s]}| map(a.$1, |b:%(ty)s| {%(gkeys)s, %(gvals)s})
)
)
"""
weld_obj.weld_code = weld_template % {"group_vec": expr.weld_code,
"gkeys": grouping_column_key_str,
"gvals": column_values_str,
"ty": tys_str,
"gty": grouping_column_ty_str}
return weld_obj
def grouped_slice(expr, type, start, size):
"""
Groups the given columns by the corresponding grouping column
value, and aggregate by summing values.
Args:
columns (List<WeldObject>): List of columns as WeldObjects
column_tys (List<str>): List of each column data ty
grouping_column (WeldObject): Column to group rest of columns by
Returns:
A WeldObject representing this computation
"""
weld_obj = WeldObject(encoder_, decoder_)
weld_obj.update(expr)
weld_template = """
map(
%(vec)s,
|b: %(type)s| {b.$0, slice(b.$1, i64(%(start)s), i64(%(size)s))}
)
"""
weld_obj.weld_code = weld_template % {"vec": expr.weld_code,
"type": type,
"start": str(start),
"size": str(size)}
return weld_obj
def get_column(columns, column_tys, index):
"""
Get column corresponding to passed-in index from ptr returned
by groupBySum.
Args:
columns (List<WeldObject>): List of columns as WeldObjects
column_tys (List<str>): List of each column data ty
index (int): index of selected column
Returns:
A WeldObject representing this computation
"""
weld_obj = WeldObject(encoder_, decoder_)
columns_var = weld_obj.update(columns, tys=WeldVec(column_tys), override=False)
if isinstance(columns, WeldObject):
columns_var = columns.obj_id
weld_obj.dependencies[columns_var] = columns
weld_template = """
map(
%(columns)s,
|elem: %(ty)s| elem.$%(index)s
)
"""
weld_obj.weld_code = weld_template % {"columns": columns_var,
"ty": column_tys,
"index": index}
return weld_obj
| weld-project/weld | python/grizzly/grizzly/grizzly_impl.py | Python | bsd-3-clause | 48,957 |
"""
Sphinx plugins for Django documentation.
"""
import json
import os
import re
from docutils import nodes
from docutils.parsers.rst import directives
from sphinx import addnodes, __version__ as sphinx_ver
from sphinx.builders.html import StandaloneHTMLBuilder
from sphinx.writers.html import SmartyPantsHTMLTranslator
from sphinx.util.console import bold
from sphinx.util.compat import Directive
from sphinx.util.nodes import set_source_info
# RE for option descriptions without a '--' prefix
simple_option_desc_re = re.compile(
r'([-_a-zA-Z0-9]+)(\s*.*?)(?=,\s+(?:/|-|--)|$)')
def setup(app):
app.add_crossref_type(
directivename="setting",
rolename="setting",
indextemplate="pair: %s; setting",
)
app.add_crossref_type(
directivename="templatetag",
rolename="ttag",
indextemplate="pair: %s; template tag"
)
app.add_crossref_type(
directivename="templatefilter",
rolename="tfilter",
indextemplate="pair: %s; template filter"
)
app.add_crossref_type(
directivename="fieldlookup",
rolename="lookup",
indextemplate="pair: %s; field lookup type",
)
app.add_description_unit(
directivename="django-admin",
rolename="djadmin",
indextemplate="pair: %s; django-admin command",
parse_node=parse_django_admin_node,
)
app.add_description_unit(
directivename="django-admin-option",
rolename="djadminopt",
indextemplate="pair: %s; django-admin command-line option",
parse_node=parse_django_adminopt_node,
)
app.add_config_value('django_next_version', '0.0', True)
app.add_directive('versionadded', VersionDirective)
app.add_directive('versionchanged', VersionDirective)
app.add_builder(DjangoStandaloneHTMLBuilder)
# register the snippet directive
app.add_directive('snippet', SnippetWithFilename)
# register a node for snippet directive so that the xml parser
# knows how to handle the enter/exit parsing event
app.add_node(snippet_with_filename,
html=(visit_snippet, depart_snippet_literal),
latex=(visit_snippet_latex, depart_snippet_latex),
man=(visit_snippet_literal, depart_snippet_literal),
text=(visit_snippet_literal, depart_snippet_literal),
texinfo=(visit_snippet_literal, depart_snippet_literal))
class snippet_with_filename(nodes.literal_block):
"""
Subclass the literal_block to override the visit/depart event handlers
"""
pass
def visit_snippet_literal(self, node):
"""
default literal block handler
"""
self.visit_literal_block(node)
def depart_snippet_literal(self, node):
"""
default literal block handler
"""
self.depart_literal_block(node)
def visit_snippet(self, node):
"""
HTML document generator visit handler
"""
lang = self.highlightlang
linenos = node.rawsource.count('\n') >= self.highlightlinenothreshold - 1
fname = node['filename']
highlight_args = node.get('highlight_args', {})
if 'language' in node:
# code-block directives
lang = node['language']
highlight_args['force'] = True
if 'linenos' in node:
linenos = node['linenos']
def warner(msg):
self.builder.warn(msg, (self.builder.current_docname, node.line))
highlighted = self.highlighter.highlight_block(node.rawsource, lang,
warn=warner,
linenos=linenos,
**highlight_args)
starttag = self.starttag(node, 'div', suffix='',
CLASS='highlight-%s' % lang)
self.body.append(starttag)
self.body.append('<div class="snippet-filename">%s</div>\n''' % (fname,))
self.body.append(highlighted)
self.body.append('</div>\n')
raise nodes.SkipNode
def visit_snippet_latex(self, node):
"""
Latex document generator visit handler
"""
self.verbatim = ''
def depart_snippet_latex(self, node):
"""
Latex document generator depart handler.
"""
code = self.verbatim.rstrip('\n')
lang = self.hlsettingstack[-1][0]
linenos = code.count('\n') >= self.hlsettingstack[-1][1] - 1
fname = node['filename']
highlight_args = node.get('highlight_args', {})
if 'language' in node:
# code-block directives
lang = node['language']
highlight_args['force'] = True
if 'linenos' in node:
linenos = node['linenos']
def warner(msg):
self.builder.warn(msg, (self.curfilestack[-1], node.line))
hlcode = self.highlighter.highlight_block(code, lang, warn=warner,
linenos=linenos,
**highlight_args)
self.body.append('\n{\\colorbox[rgb]{0.9,0.9,0.9}'
'{\\makebox[\\textwidth][l]'
'{\\small\\texttt{%s}}}}\n' % (fname,))
if self.table:
hlcode = hlcode.replace('\\begin{Verbatim}',
'\\begin{OriginalVerbatim}')
self.table.has_problematic = True
self.table.has_verbatim = True
hlcode = hlcode.rstrip()[:-14] # strip \end{Verbatim}
hlcode = hlcode.rstrip() + '\n'
self.body.append('\n' + hlcode + '\\end{%sVerbatim}\n' %
(self.table and 'Original' or ''))
self.verbatim = None
class SnippetWithFilename(Directive):
"""
The 'snippet' directive that allows to add the filename (optional)
of a code snippet in the document. This is modeled after CodeBlock.
"""
has_content = True
optional_arguments = 1
option_spec = {'filename': directives.unchanged_required}
def run(self):
code = '\n'.join(self.content)
literal = snippet_with_filename(code, code)
if self.arguments:
literal['language'] = self.arguments[0]
literal['filename'] = self.options['filename']
set_source_info(self, literal)
return [literal]
class VersionDirective(Directive):
has_content = True
required_arguments = 1
optional_arguments = 1
final_argument_whitespace = True
option_spec = {}
def run(self):
if len(self.arguments) > 1:
msg = """Only one argument accepted for directive '{directive_name}::'.
Comments should be provided as content,
not as an extra argument.""".format(directive_name=self.name)
raise self.error(msg)
env = self.state.document.settings.env
ret = []
node = addnodes.versionmodified()
ret.append(node)
if self.arguments[0] == env.config.django_next_version:
node['version'] = "Development version"
else:
node['version'] = self.arguments[0]
node['type'] = self.name
if self.content:
self.state.nested_parse(self.content, self.content_offset, node)
env.note_versionchange(node['type'], node['version'], node, self.lineno)
return ret
class DjangoHTMLTranslator(SmartyPantsHTMLTranslator):
"""
Django-specific reST to HTML tweaks.
"""
# Don't use border=1, which docutils does by default.
def visit_table(self, node):
self.context.append(self.compact_p)
self.compact_p = True
self._table_row_index = 0 # Needed by Sphinx
self.body.append(self.starttag(node, 'table', CLASS='docutils'))
def depart_table(self, node):
self.compact_p = self.context.pop()
self.body.append('</table>\n')
# <big>? Really?
def visit_desc_parameterlist(self, node):
self.body.append('(')
self.first_param = 1
self.param_separator = node.child_text_separator
def depart_desc_parameterlist(self, node):
self.body.append(')')
if sphinx_ver < '1.0.8':
#
# Don't apply smartypants to literal blocks
#
def visit_literal_block(self, node):
self.no_smarty += 1
SmartyPantsHTMLTranslator.visit_literal_block(self, node)
def depart_literal_block(self, node):
SmartyPantsHTMLTranslator.depart_literal_block(self, node)
self.no_smarty -= 1
#
# Turn the "new in version" stuff (versionadded/versionchanged) into a
# better callout -- the Sphinx default is just a little span,
# which is a bit less obvious that I'd like.
#
# FIXME: these messages are all hardcoded in English. We need to change
# that to accommodate other language docs, but I can't work out how to make
# that work.
#
version_text = {
'deprecated': 'Deprecated in Django %s',
'versionchanged': 'Changed in Django %s',
'versionadded': 'New in Django %s',
}
def visit_versionmodified(self, node):
self.body.append(
self.starttag(node, 'div', CLASS=node['type'])
)
title = "%s%s" % (
self.version_text[node['type']] % node['version'],
":" if len(node) else "."
)
self.body.append('<span class="title">%s</span> ' % title)
def depart_versionmodified(self, node):
self.body.append("</div>\n")
# Give each section a unique ID -- nice for custom CSS hooks
def visit_section(self, node):
old_ids = node.get('ids', [])
node['ids'] = ['s-' + i for i in old_ids]
node['ids'].extend(old_ids)
SmartyPantsHTMLTranslator.visit_section(self, node)
node['ids'] = old_ids
def parse_django_admin_node(env, sig, signode):
command = sig.split(' ')[0]
env._django_curr_admin_command = command
title = "django-admin.py %s" % sig
signode += addnodes.desc_name(title, title)
return sig
def parse_django_adminopt_node(env, sig, signode):
"""A copy of sphinx.directives.CmdoptionDesc.parse_signature()"""
from sphinx.domains.std import option_desc_re
count = 0
firstname = ''
for m in option_desc_re.finditer(sig):
optname, args = m.groups()
if count:
signode += addnodes.desc_addname(', ', ', ')
signode += addnodes.desc_name(optname, optname)
signode += addnodes.desc_addname(args, args)
if not count:
firstname = optname
count += 1
if not count:
for m in simple_option_desc_re.finditer(sig):
optname, args = m.groups()
if count:
signode += addnodes.desc_addname(', ', ', ')
signode += addnodes.desc_name(optname, optname)
signode += addnodes.desc_addname(args, args)
if not count:
firstname = optname
count += 1
if not firstname:
raise ValueError
return firstname
class DjangoStandaloneHTMLBuilder(StandaloneHTMLBuilder):
"""
Subclass to add some extra things we need.
"""
name = 'djangohtml'
def finish(self):
super(DjangoStandaloneHTMLBuilder, self).finish()
self.info(bold("writing templatebuiltins.js..."))
xrefs = self.env.domaindata["std"]["objects"]
templatebuiltins = {
"ttags": [n for ((t, n), (l, a)) in xrefs.items()
if t == "templatetag" and l == "ref/templates/builtins"],
"tfilters": [n for ((t, n), (l, a)) in xrefs.items()
if t == "templatefilter" and l == "ref/templates/builtins"],
}
outfilename = os.path.join(self.outdir, "templatebuiltins.js")
with open(outfilename, 'w') as fp:
fp.write('var django_template_builtins = ')
json.dump(templatebuiltins, fp)
fp.write(';\n')
| Beeblio/django | docs/_ext/djangodocs.py | Python | bsd-3-clause | 11,807 |
# -*- coding: utf-8 -*-
# Generated by Django 1.11.13 on 2018-06-01 12:16
from __future__ import unicode_literals
from django.db import migrations, models
class Migration(migrations.Migration):
dependencies = [("contentstore", "0007_auto_20171102_0950")]
operations = [
migrations.AddField(
model_name="schedule",
name="scheduler_schedule_id",
field=models.UUIDField(
blank=True,
default=None,
null=True,
verbose_name="scheduler schedule id",
),
)
]
| praekelt/seed-stage-based-messaging | contentstore/migrations/0008_schedule_scheduler_schedule_id.py | Python | bsd-3-clause | 595 |
from django import forms
from panoptes.core.utils.registry import create_registry
ChartRegistry = create_registry('slug')
class BaseChart(object):
"""Abstract base class for a chart.
A child chart must define a string value for the `slug` attribute, and can
optionally define values for the `js` and `css` attributes, which should be
structured in the same way as the attributes of a Django Media class.
"""
__metaclass__ = ChartRegistry
slug = None
template = None
def __init__(self, sessions):
"""
Create a chart for the data contained in the FilteredSessions instance
`sessions`.
"""
self.sessions = sessions
def provide_render_args(self):
"""Allow a chart to return render arguments.."""
return {}
@property
def media(self):
return forms.Media(getattr(self, 'Media', None))
| cilcoberlin/panoptes | panoptes/analysis/panels/charting/charts/base.py | Python | bsd-3-clause | 816 |
import collections
print "\n\n[INFO] Processing articles for publication venues.\n"
print "------------------------------------------------------------------------------------------------\n\n"
file = open("../data/aminer_publication_tiny.txt")
lines = file.readlines()
papers = {}
i = 0
article_count = 0
pub_count = 0
author_list_temp = []
author_article_count = collections.OrderedDict()
author = set()
while i < len(lines) :
if lines[i].startswith('#@') : | sujithvm/internationality-journals | src/test.py | Python | mit | 464 |
#!/usr/bin/python
# -*- coding: utf-8 -*-
import __init__
import settings
import random
from bson import ObjectId
class Guild(settings.BaseObj):
data = {
'name': '',
'search_name': '',
'link_name': '',
'description':'',
'people':[],
'creator': '',
'img': 'none',
'score': 0,
'open': 1,
'people_count':0,
'request': [],
'site_link': '',
'pvp_score' : 0,
'faction': -1,
'guild_points': 0
}
class GuildNews(settings.BaseObj):
data = {
'guild_id': 0,
'news': []
}
class ModelGuilds():
col_guilds = settings.database.cols['guilds']
col_players = settings.database.cols['players']
col_gmessages = settings.database.cols['col_guild_messages']
col_gnews = settings.database.cols['col_guilds_news']
def __init__(self, connection):
self.mongo = connection
self.balance = settings.balance
# GET
def getPlayersGuild(self, user_id):
return self.mongo.find(self.col_guilds, {'people':{'$all':[user_id]}})
def getTopGuildsByPeopleCount(self, count = 10):
return self.mongo.getu(self.col_guilds, {}, fields = {'name':1, 'people_count':1, 'img':1, 'pvp_score':1}, limit = count, sort = {'people_count': -1})
def getGuildByName(self, name, type_of_name='search_name'):
if type_of_name == 'search_name':
name = name.upper()
return self.mongo.find(self.col_guilds, {type_of_name: name})
def getGuildMembers(self, guild, field = 'people', sort_query = {}):
if not field in guild or not guild[field]:
return []
players = self.mongo.findSomeIDs(self.col_players, guild[field], sort=sort_query, fields={
'_id': 1,
'name': 1,
'class': 1,
'lvl':1,
#'stat':1,
'avatar': 1,
'achv_points': 1,
'pvp_score': 1,
'race': 1,
'faction': 1,
'user_id': 1,
'stat.lead.current': 1
})
return players
def getGuildNews(self, guild_id):
return self.mongo.find(self.col_gnews, {'guild_id': guild_id})
def getGuildToWarList(self, my_guild_name, limit = 10):
return self.mongo.getu(self.col_guilds, search={'name': {'$ne': my_guild_name}}, limit=limit)
def getGuildByID(self, id):
return self.mongo.find(self.col_guilds, {'_id': id})
def getGuildsByIDs(self, ids):
return self.mongo.findSomeIDs(self.col_guilds, ids, fields={
'_id': 1,
'name': 1,
})
def getGuildMessages(self, guild_name):
return self.mongo.find(self.col_gmessages, {'name': guild_name})
def getGuilds(self):
return self.mongo.getu(self.col_guilds, fields={
"pvp_score": 1,
"people": 1,
"faction": 1,
"score": 1,
"people_count": 1,
"name": 1
})
def getTrendingGuilds(self, limit = 10):
people_count = random.randint(5, 35)
pvp_score = random.randint(1,1000)
gte = random.randint(0,1)
return self.mongo.getu(
self.col_guilds,
search={
'people_count': {['$gte','$lte'][gte]: people_count},
'pvp_score': {'$gte':pvp_score},
},
fields={'name':1, 'people_count':1, 'img':1, 'link_name':1, 'pvp_score':1},
limit=limit
)
def getGuildsListFiltered(self, count = 10, search_query = {}, page = 1, field = False, sort = -1):
if field:
sort_query = {field: sort}
else:
sort_query = {}
fields = {'name':1, 'img':1, 'people_count':1, 'pvp_score':1, 'guild_points': 1}
return self.mongo.getu(self.col_guilds, search = search_query, fields = fields, skip = count*(page-1), limit = count, sort = sort_query)
def getGuildsListSearch(self, search_query):
return self.mongo.getu(self.col_guilds, search_query, {'name': 1, '_id': 0}, limit=10)
def getGuildsCount(self):
return self.mongo.count(self.col_guilds)
def getTopPvPGuildPlayers(self, guild_id, min_pvp_score = 0, count = 5):
guild_people = self.mongo.find(self.col_guilds, {'_id': guild_id}, {'people': 1})
if guild_people:
query = []
for id in guild_people['people']:
query.append({'_id':id})
if query:
return self.mongo.getu(
self.col_players,
search={"$or" : query},
fields={'_id': 1, 'name': 1},
sort={'pvp_score': -1},
limit=count
)
return False
# ADD
def addGuild(self, guild):
player_id = guild['creator']
if self.mongo.find(self.col_guilds, {'search_name': guild['search_name']}):
return False
self.mongo.insert(self.col_guilds, guild)
self.mongo.update(self.col_players, {'_id': player_id}, {'_guild_name': guild['name']})
history = [self.balance.guild_message]
record = {'name': guild['name'], 'history': history}
self.mongo.insert(self.col_gmessages, record)
guild_id = self.mongo.find(self.col_guilds, {'name': guild['name']}, {'_id':1})['_id']
self.mongo.insert(self.col_gnews, {
'guild_id': guild_id,
'news': []
})
return True
# MISC
def rawUpdateGuild(self, guild_name, fields):
self.mongo.raw_update(self.col_guilds, {'name': guild_name}, fields)
def setGuildMessages(self, guild_name, messages):
self.mongo.update(self.col_gmessages, {'name': guild_name}, {'history': messages})
def clearGuildSettings(self, player_id):
self.mongo.update(self.col_players, {'_id': player_id}, {'_guild_name': ''})
def changeSettings(self, guild_id, settings):
self.mongo.update(self.col_guilds, {'_id': guild_id}, settings)
def leaveGuild(self, guild_id, player_id):
self.mongo.raw_update(self.col_guilds, {'_id': guild_id}, {'$inc':{'people_count': -1}, "$pull":{'people': player_id}})
self.clearGuildSettings(player_id)
def joinGuild(self, guild_id, player_id, guild_name = ''):
self.mongo.update(self.col_players, {'_id': player_id}, {'_guild_name': guild_name})
self.mongo.raw_update(self.col_guilds, {'_id': guild_id}, {'$inc':{'people_count': 1}, "$push":{'people': player_id}})
def isGuildExist(self, search_name):
return self.mongo.find(self.col_guilds, {'search_name': search_name})
def promoteLeadership(self, guild_id, player_name):
player = self.mongo.find(self.col_players, {'name': player_name}, {'_id':1})
if player:
self.mongo.update(self.col_guilds, {'_id': guild_id}, {'creator':player['_id']})
def removeGuild(self, guild_id, player_id):
self.mongo.remove(self.col_guilds, {'_id': guild_id})
self.mongo.remove(self.col_gnews, {'guild_id': guild_id})
self.clearGuildSettings(player_id)
def askInvite(self, guild_id, player_id):
self.mongo.raw_update(self.col_guilds, {'_id': guild_id}, {"$push":{'request': player_id}})
def rejectInvite(self, guild_id, player_id):
self.mongo.raw_update(self.col_guilds, {'_id': guild_id}, {"$pull":{'request': player_id}})
def removeAllRequests(self, player_id):
guilds = self.mongo.getu(self.col_guilds, { 'request': {'$all': [player_id]}}, {'_id':1})
for guild in guilds:
self.mongo.raw_update(self.col_guilds, {'_id': guild['_id']}, {"$pull":{'request': player_id}})
# NEWS
def getGuildNews(self, guild_id):
return self.mongo.find(self.col_gnews, {'guild_id': guild_id})
def addNewsToBase(self, guild_id, news_body):
self.mongo.raw_update(self.col_gnews, {'guild_id': guild_id}, {'$push': {'news': news_body}})
print self.mongo.getLastError()
def removeNewsFromBase(self, guild_id, news_uid):
self.mongo.raw_update(self.col_gnews, {'guild_id': guild_id}, {'$pull': {'news': {'UID': news_uid} }})
| idooo/tweeria | models/model_guilds.py | Python | mit | 7,396 |
# dev server settings for core project.
from base import *
DEBUG = True
DATABASES = {
'default': {
# The last part of ENGINE is 'postgresql_psycopg2', 'mysql', 'sqlite3' or 'ado_mssql'.
'ENGINE': 'django.db.backends.postgresql_psycopg2',
'NAME': '', # Or path to database file if using sqlite3
'USER': '', # Not used with sqlite3.
'PASSWORD': '', # Not used with sqlite3.
'HOST': '', # Set to empty string for localhost. Not used with sqlite3.
'PORT': '', # Set to empty string for default. Not used with sqlite3.
'SCHEMA': '',
}
} | mrkeng/fabric-bolt | core/settings/develop.py | Python | mit | 650 |
# Copyright (c) Twisted Matrix Laboratories.
# See LICENSE for details.
"""
Twisted Python: Utilities and Enhancements for Python.
"""
from __future__ import absolute_import, division
# Deprecating twisted.python.constants.
from .compat import unicode
from .versions import Version
from .deprecate import deprecatedModuleAttribute
deprecatedModuleAttribute(
Version("Twisted", 16, 5, 0),
"Please use constantly from PyPI instead.",
"twisted.python", "constants")
deprecatedModuleAttribute(
Version('Twisted', 17, 5, 0),
"Please use hyperlink from PyPI instead.",
"twisted.python", "url")
del Version
del deprecatedModuleAttribute
del unicode
| whitehorse-io/encarnia | pyenv/lib/python2.7/site-packages/twisted/python/__init__.py | Python | mit | 674 |
import functools
import operator
import os
import re
try:
import simplejson as json
except ImportError:
import json
from flask import Blueprint
from flask import Response
from flask import abort
from flask import flash
from flask import redirect
from flask import render_template
from flask import request
from flask import session
from flask import url_for
from flask_peewee.filters import FilterForm
from flask_peewee.filters import FilterMapping
from flask_peewee.filters import FilterModelConverter
from flask_peewee.forms import BaseModelConverter
from flask_peewee.forms import ChosenAjaxSelectWidget
from flask_peewee.forms import LimitedModelSelectField
from flask_peewee.serializer import Serializer
from flask_peewee.utils import PaginatedQuery
from flask_peewee.utils import get_next
from flask_peewee.utils import path_to_models
from flask_peewee.utils import slugify
from peewee import BooleanField
from peewee import DateField
from peewee import DateTimeField
from peewee import ForeignKeyField
from peewee import TextField
from werkzeug import Headers
from wtforms import fields
from wtforms import widgets
from wtfpeewee.fields import ModelHiddenField
from wtfpeewee.fields import ModelSelectField
from wtfpeewee.fields import ModelSelectMultipleField
from wtfpeewee.orm import model_form
current_dir = os.path.dirname(__file__)
class AdminModelConverter(BaseModelConverter):
def __init__(self, model_admin, additional=None):
super(AdminModelConverter, self).__init__(additional)
self.model_admin = model_admin
def handle_foreign_key(self, model, field, **kwargs):
if field.null:
kwargs['allow_blank'] = True
if field.name in (self.model_admin.foreign_key_lookups or ()):
form_field = ModelHiddenField(model=field.rel_model, **kwargs)
else:
form_field = ModelSelectField(model=field.rel_model, **kwargs)
return field.name, form_field
class AdminFilterModelConverter(FilterModelConverter):
def __init__(self, model_admin, additional=None):
super(AdminFilterModelConverter, self).__init__(additional)
self.model_admin = model_admin
def handle_foreign_key(self, model, field, **kwargs):
if field.name in (self.model_admin.foreign_key_lookups or ()):
data_source = url_for(self.model_admin.get_url_name('ajax_list'))
widget = ChosenAjaxSelectWidget(data_source, field.name)
form_field = LimitedModelSelectField(model=field.rel_model, widget=widget, **kwargs)
else:
form_field = ModelSelectField(model=field.rel_model, **kwargs)
return field.name, form_field
class ModelAdmin(object):
"""
ModelAdmin provides create/edit/delete functionality for a peewee Model.
"""
paginate_by = 20
filter_paginate_by = 15
# columns to display in the list index - can be field names or callables on
# a model instance, though in the latter case they will not be sortable
columns = None
# exclude certian fields from being exposed as filters -- for related fields
# use "__" notation, e.g. user__password
filter_exclude = None
filter_fields = None
# form parameters, lists of fields
exclude = None
fields = None
form_converter = AdminModelConverter
# foreign_key_field --> related field to search on, e.g. {'user': 'username'}
foreign_key_lookups = None
# delete behavior
delete_collect_objects = True
delete_recursive = True
filter_mapping = FilterMapping
filter_converter = AdminFilterModelConverter
# templates, to override see get_template_overrides()
base_templates = {
'index': 'admin/models/index.html',
'add': 'admin/models/add.html',
'edit': 'admin/models/edit.html',
'delete': 'admin/models/delete.html',
'export': 'admin/models/export.html',
}
def __init__(self, admin, model):
self.admin = admin
self.model = model
self.db = model._meta.database
self.pk = self.model._meta.primary_key
self.templates = dict(self.base_templates)
self.templates.update(self.get_template_overrides())
def get_template_overrides(self):
return {}
def get_url_name(self, name):
return '%s.%s_%s' % (
self.admin.blueprint.name,
self.get_admin_name(),
name,
)
def get_filter_form(self):
return FilterForm(
self.model,
self.filter_converter(self),
self.filter_mapping(),
self.filter_fields,
self.filter_exclude,
)
def process_filters(self, query):
filter_form = self.get_filter_form()
form, query, cleaned = filter_form.process_request(query)
return form, query, cleaned, filter_form._field_tree
def get_form(self, adding=False):
allow_pk = adding and not self.model._meta.auto_increment
return model_form(self.model,
allow_pk=allow_pk,
only=self.fields,
exclude=self.exclude,
converter=self.form_converter(self),
)
def get_add_form(self):
return self.get_form(adding=True)
def get_edit_form(self, instance):
return self.get_form()
def get_query(self):
return self.model.select()
def get_object(self, pk):
return self.get_query().where(self.pk==pk).get()
def get_urls(self):
return (
('/', self.index),
('/add/', self.add),
('/delete/', self.delete),
('/export/', self.export),
('/<pk>/', self.edit),
('/_ajax/', self.ajax_list),
)
def get_columns(self):
return self.model._meta.sorted_field_names
def column_is_sortable(self, col):
return col in self.model._meta.fields
def get_display_name(self):
return self.model.__name__
def get_admin_name(self):
return slugify(self.model.__name__)
def save_model(self, instance, form, adding=False):
form.populate_obj(instance)
instance.save(force_insert=adding)
return instance
def apply_ordering(self, query, ordering):
if ordering:
desc, column = ordering.startswith('-'), ordering.lstrip('-')
if self.column_is_sortable(column):
field = self.model._meta.fields[column]
query = query.order_by(field.asc() if not desc else field.desc())
return query
def get_extra_context(self):
return {}
def index(self):
session['%s.index' % self.get_admin_name()] = request.url
query = self.get_query()
ordering = request.args.get('ordering') or ''
query = self.apply_ordering(query, ordering)
# process the filters from the request
filter_form, query, cleaned, field_tree = self.process_filters(query)
# create a paginated query out of our filtered results
pq = PaginatedQuery(query, self.paginate_by)
if request.method == 'POST':
id_list = request.form.getlist('id')
if request.form['action'] == 'delete':
return redirect(url_for(self.get_url_name('delete'), id=id_list))
else:
return redirect(url_for(self.get_url_name('export'), id=id_list))
return render_template(self.templates['index'],
model_admin=self,
query=pq,
ordering=ordering,
filter_form=filter_form,
field_tree=field_tree,
active_filters=cleaned,
**self.get_extra_context()
)
def dispatch_save_redirect(self, instance):
if 'save' in request.form:
url = (session.get('%s.index' % self.get_admin_name()) or
url_for(self.get_url_name('index')))
return redirect(url)
elif 'save_add' in request.form:
return redirect(url_for(self.get_url_name('add')))
else:
return redirect(
url_for(self.get_url_name('edit'), pk=instance.get_id())
)
def add(self):
Form = self.get_add_form()
instance = self.model()
if request.method == 'POST':
form = Form(request.form)
if form.validate():
instance = self.save_model(instance, form, True)
flash('New %s saved successfully' % self.get_display_name(), 'success')
return self.dispatch_save_redirect(instance)
else:
form = Form()
return render_template(self.templates['add'],
model_admin=self,
form=form,
instance=instance,
**self.get_extra_context()
)
def edit(self, pk):
try:
instance = self.get_object(pk)
except self.model.DoesNotExist:
abort(404)
Form = self.get_edit_form(instance)
if request.method == 'POST':
form = Form(request.form, obj=instance)
if form.validate():
self.save_model(instance, form, False)
flash('Changes to %s saved successfully' % self.get_display_name(), 'success')
return self.dispatch_save_redirect(instance)
else:
form = Form(obj=instance)
return render_template(self.templates['edit'],
model_admin=self,
instance=instance,
form=form,
**self.get_extra_context()
)
def collect_objects(self, obj):
deps = obj.dependencies()
objects = []
for query, fk in obj.dependencies():
if not fk.null:
sq = fk.model_class.select().where(query)
collected = [rel_obj for rel_obj in sq.execute().iterator()]
if collected:
objects.append((0, fk.model_class, collected))
return sorted(objects, key=lambda i: (i[0], i[1].__name__))
def delete(self):
if request.method == 'GET':
id_list = request.args.getlist('id')
else:
id_list = request.form.getlist('id')
query = self.model.select().where(self.pk << id_list)
if request.method == 'GET':
collected = {}
if self.delete_collect_objects:
for obj in query:
collected[obj.get_id()] = self.collect_objects(obj)
elif request.method == 'POST':
count = query.count()
for obj in query:
obj.delete_instance(recursive=self.delete_recursive)
flash('Successfully deleted %s %ss' % (count, self.get_display_name()), 'success')
url = (session.get('%s.index' % self.get_admin_name()) or
url_for(self.get_url_name('index')))
return redirect(url)
return render_template(self.templates['delete'], **dict(
model_admin=self,
query=query,
collected=collected,
**self.get_extra_context()
))
def collect_related_fields(self, model, accum, path, seen=None):
seen = seen or set()
path_str = '__'.join(path)
for field in model._meta.sorted_fields:
if isinstance(field, ForeignKeyField) and field not in seen:
seen.add(field)
self.collect_related_fields(field.rel_model, accum, path + [field.name], seen)
elif model != self.model:
accum.setdefault((model, path_str), [])
accum[(model, path_str)].append(field)
return accum
def export(self):
query = self.get_query()
ordering = request.args.get('ordering') or ''
query = self.apply_ordering(query, ordering)
# process the filters from the request
filter_form, query, cleaned, field_tree = self.process_filters(query)
related = self.collect_related_fields(self.model, {}, [])
# check for raw id
id_list = request.args.getlist('id')
if id_list:
query = query.where(self.pk << id_list)
if request.method == 'POST':
raw_fields = request.form.getlist('fields')
export = Export(query, related, raw_fields)
return export.json_response('export-%s.json' % self.get_admin_name())
return render_template(self.templates['export'],
model_admin=self,
model=query.model_class,
query=query,
filter_form=filter_form,
field_tree=field_tree,
active_filters=cleaned,
related_fields=related,
sql=query.sql(),
**self.get_extra_context()
)
def ajax_list(self):
field_name = request.args.get('field')
prev_page = 0
next_page = 0
try:
models = path_to_models(self.model, field_name)
except AttributeError:
data = []
else:
field = self.model._meta.fields[field_name]
rel_model = models.pop()
rel_field = rel_model._meta.fields[self.foreign_key_lookups[field_name]]
query = rel_model.select().order_by(rel_field)
query_string = request.args.get('query')
if query_string:
query = query.where(rel_field ** ('%%%s%%' % query_string))
pq = PaginatedQuery(query, self.filter_paginate_by)
current_page = pq.get_page()
if current_page > 1:
prev_page = current_page - 1
if current_page < pq.get_pages():
next_page = current_page + 1
data = []
# if the field is nullable, include the "None" option at the top of the list
if field.null:
data.append({'id': '__None', 'repr': 'None'})
data.extend([{'id': obj.get_id(), 'repr': unicode(obj)} for obj in pq.get_list()])
json_data = json.dumps({'prev_page': prev_page, 'next_page': next_page, 'object_list': data})
return Response(json_data, mimetype='application/json')
class AdminPanel(object):
template_name = 'admin/panels/default.html'
def __init__(self, admin, title):
self.admin = admin
self.title = title
self.slug = slugify(self.title)
def dashboard_url(self):
return url_for('%s.index' % (self.admin.blueprint.name))
def get_urls(self):
return ()
def get_url_name(self, name):
return '%s.panel_%s_%s' % (
self.admin.blueprint.name,
self.slug,
name,
)
def get_template_name(self):
return self.template_name
def get_context(self):
return {}
def render(self):
return render_template(self.get_template_name(), panel=self, **self.get_context())
class AdminTemplateHelper(object):
def __init__(self, admin):
self.admin = admin
self.app = self.admin.app
def get_model_field(self, model, field):
try:
attr = getattr(model, field)
except AttributeError:
model_admin = self.admin[type(model)]
try:
attr = getattr(model_admin, field)
except AttributeError:
raise AttributeError('Could not find attribute or method '
'named "%s".' % field)
else:
return attr(model)
else:
if callable(attr):
attr = attr()
return attr
def get_form_field(self, form, field_name):
return getattr(form, field_name)
def fix_underscores(self, s):
return s.replace('_', ' ').title()
def update_querystring(self, querystring, key, val):
if not querystring:
return '%s=%s' % (key, val)
else:
querystring = re.sub('%s(?:[^&]+)?&?' % key, '', querystring.decode('utf8')).rstrip('&')
return ('%s&%s=%s' % (querystring, key, val)).lstrip('&')
def get_verbose_name(self, model, column_name):
try:
field = model._meta.fields[column_name]
except KeyError:
return self.fix_underscores(column_name)
else:
return field.verbose_name
def get_model_admins(self):
return {'model_admins': self.admin.get_model_admins(), 'branding': self.admin.branding}
def get_admin_url(self, obj):
model_admin = self.admin.get_admin_for(type(obj))
if model_admin:
return url_for(model_admin.get_url_name('edit'), pk=obj.get_id())
def get_model_name(self, model_class):
model_admin = self.admin.get_admin_for(model_class)
if model_admin:
return model_admin.get_display_name()
return model_class.__name__
def apply_prefix(self, field_name, prefix_accum, field_prefix, rel_prefix='fr_', rel_sep='-'):
accum = []
for prefix in prefix_accum:
accum.append('%s%s' % (rel_prefix, prefix))
accum.append('%s%s' % (field_prefix, field_name))
return rel_sep.join(accum)
def prepare_environment(self):
self.app.template_context_processors[None].append(self.get_model_admins)
self.app.jinja_env.globals['get_model_field'] = self.get_model_field
self.app.jinja_env.globals['get_form_field'] = self.get_form_field
self.app.jinja_env.globals['get_verbose_name'] = self.get_verbose_name
self.app.jinja_env.filters['fix_underscores'] = self.fix_underscores
self.app.jinja_env.globals['update_querystring'] = self.update_querystring
self.app.jinja_env.globals['get_admin_url'] = self.get_admin_url
self.app.jinja_env.globals['get_model_name'] = self.get_model_name
self.app.jinja_env.filters['apply_prefix'] = self.apply_prefix
class Admin(object):
def __init__(self, app, auth, template_helper=AdminTemplateHelper,
prefix='/admin', name='admin', branding='flask-peewee'):
self.app = app
self.auth = auth
self._admin_models = {}
self._registry = {}
self._panels = {}
self.blueprint = self.get_blueprint(name)
self.url_prefix = prefix
self.template_helper = template_helper(self)
self.template_helper.prepare_environment()
self.branding = branding
def auth_required(self, func):
@functools.wraps(func)
def inner(*args, **kwargs):
user = self.auth.get_logged_in_user()
if not user:
login_url = url_for('%s.login' % self.auth.blueprint.name, next=get_next())
return redirect(login_url)
if not self.check_user_permission(user):
abort(403)
return func(*args, **kwargs)
return inner
def check_user_permission(self, user):
return user.admin
def get_urls(self):
return (
('/', self.auth_required(self.index)),
)
def __contains__(self, item):
return item in self._registry
def __getitem__(self, item):
return self._registry[item]
def register(self, model, admin_class=ModelAdmin):
model_admin = admin_class(self, model)
admin_name = model_admin.get_admin_name()
self._registry[model] = model_admin
def unregister(self, model):
del(self._registry[model])
def register_panel(self, title, panel):
panel_instance = panel(self, title)
self._panels[title] = panel_instance
def unregister_panel(self, title):
del(self._panels[title])
def get_admin_for(self, model):
return self._registry.get(model)
def get_model_admins(self):
return sorted(self._registry.values(), key=lambda o: o.get_admin_name())
def get_panels(self):
return sorted(self._panels.values(), key=lambda o: o.slug)
def index(self):
return render_template('admin/index.html',
model_admins=self.get_model_admins(),
panels=self.get_panels(),
)
def get_blueprint(self, blueprint_name):
return Blueprint(
blueprint_name,
__name__,
static_folder=os.path.join(current_dir, 'static'),
template_folder=os.path.join(current_dir, 'templates'),
)
def register_blueprint(self, **kwargs):
self.app.register_blueprint(
self.blueprint,
url_prefix=self.url_prefix,
**kwargs
)
def configure_routes(self):
for url, callback in self.get_urls():
self.blueprint.route(url, methods=['GET', 'POST'])(callback)
for model_admin in self._registry.values():
admin_name = model_admin.get_admin_name()
for url, callback in model_admin.get_urls():
full_url = '/%s%s' % (admin_name, url)
self.blueprint.add_url_rule(
full_url,
'%s_%s' % (admin_name, callback.__name__),
self.auth_required(callback),
methods=['GET', 'POST'],
)
for panel in self._panels.values():
for url, callback in panel.get_urls():
full_url = '/%s%s' % (panel.slug, url)
self.blueprint.add_url_rule(
full_url,
'panel_%s_%s' % (panel.slug, callback.__name__),
self.auth_required(callback),
methods=['GET', 'POST'],
)
def setup(self):
self.configure_routes()
self.register_blueprint()
class Export(object):
def __init__(self, query, related, fields):
self.query = query
self.related = related
self.fields = fields
self.alias_to_model = dict([(k[1], k[0]) for k in self.related.keys()])
def prepare_query(self):
clone = self.query.clone()
select = []
joined = set()
def ensure_join(query, m, p):
if m not in joined:
if '__' not in p:
next_model = query.model_class
else:
next, _ = p.rsplit('__', 1)
next_model = self.alias_to_model[next]
query = ensure_join(query, next_model, next)
joined.add(m)
return query.switch(next_model).join(m)
else:
return query
for lookup in self.fields:
# lookup may be something like "content" or "user__user_name"
if '__' in lookup:
path, column = lookup.rsplit('__', 1)
model = self.alias_to_model[path]
clone = ensure_join(clone, model, path)
else:
model = self.query.model_class
column = lookup
field = model._meta.fields[column]
select.append(field)
clone._select = select
return clone
def json_response(self, filename='export.json'):
serializer = Serializer()
prepared_query = self.prepare_query()
field_dict = {}
for field in prepared_query._select:
field_dict.setdefault(field.model_class, [])
field_dict[field.model_class].append(field.name)
def generate():
i = prepared_query.count()
yield '[\n'
for obj in prepared_query:
i -= 1
yield json.dumps(serializer.serialize_object(obj, field_dict))
if i > 0:
yield ',\n'
yield '\n]'
headers = Headers()
headers.add('Content-Type', 'application/javascript')
headers.add('Content-Disposition', 'attachment; filename=%s' % filename)
return Response(generate(), mimetype='text/javascript', headers=headers, direct_passthrough=True)
| ariakerstein/twitterFlaskClone | project/lib/python2.7/site-packages/flask_peewee/admin.py | Python | mit | 23,963 |
## -*- coding: utf-8 -*-
# (C) 2013 Muthiah Annamalai
#
# Implementation of transliteration algorithm flavors
# and later used in TamilKaruvi (2007) by your's truly.
#
# BlindIterative Algorithm from TamilKaruvi - less than optimal -
class BlindIterative:
@staticmethod
def transliterate(table,english_str):
""" @table - has to be one of Jaffna or Azhagi etc.
@english_str - """
#
# -details-
#
# While text remains:
# Lookup the English part in anywhere in the string position
# If present:
# Lookup the Corresponding Tamil Part & Append it to the string.
# Else:
# Continue
#
out_str = english_str
# for consistent results we need to work on sorted keys
eng_parts = list(table.keys())
eng_parts.sort()
eng_parts.reverse()
for eng_part in eng_parts:
tamil_equiv = table[eng_part]
parts = out_str.split( eng_part )
out_str = tamil_equiv.join( parts )
return out_str
# Azhagi has a many-to-one mapping - using a Tamil language model and Baye's conditional probabilities
# to break the tie when two or more Tamil letters have the same English syllable. Currently
# this predictive transliterator is not available in this package. Also such an algorithm could be
# used with any table.
#
# challenge use a probabilistic model on Tamil language to score the next letter,
# instead of using the longest/earliest match
# http://www.mazhalaigal.com/tamil/learn/keys.php
class Predictive:
@staticmethod
def transliterate(table,english_str):
raise Exception("Not Implemented!")
pass
# Sequential Iterative Algorithm modified from TamilKaruvi
class Iterative:
@staticmethod
def transliterate(table,english_str):
""" @table - has to be one of Jaffna or Azhagi etc.
@english_str - """
#
# -details-
#
# While text remains:
# Lookup the First-Matching-Longest-English part
# If present:
# Lookup the Corresponding Tamil Part & Append it.
# Else:
# Continue
#
out_str = english_str
# for consistent results we need to work on sorted keys
eng_parts = list(table.keys())
eng_parts.sort()
eng_parts.reverse()
MAX_ITERS_BEFORE_YOU_DROP_LETTER = max(list(map(len,eng_parts)))
remaining = len(english_str)
out_str = ''
pos = 0
while pos < remaining:
# try to find the longest prefix in input from L->R in greedy fashion
iters = MAX_ITERS_BEFORE_YOU_DROP_LETTER
success = False
while iters >= 0:
curr_prefix = english_str[pos:min(pos+iters-1,remaining)]
curr_prefix_lower = None
if ( len(curr_prefix) >= 2 ):
curr_prefix_lower = curr_prefix[0].lower() + curr_prefix[1:]
## print curr_prefix
iters = iters - 1
if ( curr_prefix in eng_parts ):
out_str = out_str + table[curr_prefix]
pos = pos + len( curr_prefix)
success = True
break;
elif ( curr_prefix_lower in eng_parts ):
out_str = out_str + table[curr_prefix_lower]
pos = pos + len( curr_prefix_lower )
success = True
break;
# replacement was a success
if ( success ):
continue
# too-bad we didn't find a replacement - just copy char to output
## print "concatennate the unmatched =>",english_str[pos],"<="
if ord(english_str[pos]) < 128:
rep_char = english_str[pos]
else:
rep_char = "?"
out_str = out_str + rep_char
pos = pos + 1
return out_str
| atvKumar/open-tamil | transliterate/algorithm.py | Python | mit | 4,159 |
#!/usr/bin/python
# -*- coding: utf-8 -*-
"""
An incomplete sample script.
This is not a complete bot; rather, it is a template from which simple
bots can be made. You can rename it to mybot.py, then edit it in
whatever way you want.
Use global -simulate option for test purposes. No changes to live wiki
will be done.
The following parameters are supported:
¶ms;
-always The bot won't ask for confirmation when putting a page
-text: Use this text to be added; otherwise 'Test' is used
-replace: Dont add text but replace it
-top Place additional text on top of the page
-summary: Set the action summary message for the edit.
"""
#
# (C) Pywikibot team, 2006-2017
#
# Distributed under the terms of the MIT license.
#
from __future__ import absolute_import, unicode_literals
__version__ = '$Id$'
#
import pywikibot
from pywikibot import pagegenerators
from pywikibot.bot import (
SingleSiteBot, ExistingPageBot, NoRedirectPageBot, AutomaticTWSummaryBot)
from pywikibot.tools import issue_deprecation_warning
# This is required for the text that is shown when you run this script
# with the parameter -help.
docuReplacements = {
'¶ms;': pagegenerators.parameterHelp
}
class BasicBot(
# Refer pywikobot.bot for generic bot classes
SingleSiteBot, # A bot only working on one site
# CurrentPageBot, # Sets 'current_page'. Process it in treat_page method.
# # Not needed here because we have subclasses
ExistingPageBot, # CurrentPageBot which only treats existing pages
NoRedirectPageBot, # CurrentPageBot which only treats non-redirects
AutomaticTWSummaryBot, # Automatically defines summary; needs summary_key
):
"""
An incomplete sample bot.
@ivar summary_key: Edit summary message key. The message that should be used
is placed on /i18n subdirectory. The file containing these messages
should have the same name as the caller script (i.e. basic.py in this
case). Use summary_key to set a default edit summary message.
@type summary_key: str
"""
summary_key = 'basic-changing'
def __init__(self, generator, **kwargs):
"""
Constructor.
@param generator: the page generator that determines on which pages
to work
@type generator: generator
"""
# Add your own options to the bot and set their defaults
# -always option is predefined by BaseBot class
self.availableOptions.update({
'replace': False, # delete old text and write the new text
'summary': None, # your own bot summary
'text': 'Test', # add this text from option. 'Test' is default
'top': False, # append text on top of the page
})
# call constructor of the super class
super(BasicBot, self).__init__(site=True, **kwargs)
# handle old -dry parameter
self._handle_dry_param(**kwargs)
# assign the generator to the bot
self.generator = generator
def _handle_dry_param(self, **kwargs):
"""
Read the dry parameter and set the simulate variable instead.
This is a private method. It prints a deprecation warning for old
-dry paramter and sets the global simulate variable and informs
the user about this setting.
The constuctor of the super class ignores it because it is not
part of self.availableOptions.
@note: You should ommit this method in your own application.
@keyword dry: deprecated option to prevent changes on live wiki.
Use -simulate instead.
@type dry: bool
"""
if 'dry' in kwargs:
issue_deprecation_warning('dry argument',
'pywikibot.config.simulate', 1)
# use simulate variable instead
pywikibot.config.simulate = True
pywikibot.output('config.simulate was set to True')
def treat_page(self):
"""Load the given page, do some changes, and save it."""
text = self.current_page.text
################################################################
# NOTE: Here you can modify the text in whatever way you want. #
################################################################
# If you find out that you do not want to edit this page, just return.
# Example: This puts Text on a page.
# Retrieve your private option
# Use your own text or use the default 'Test'
text_to_add = self.getOption('text')
if self.getOption('replace'):
# replace the page text
text = text_to_add
elif self.getOption('top'):
# put text on top
text = text_to_add + text
else:
# put text on bottom
text += text_to_add
# if summary option is None, it takes the default i18n summary from
# i18n subdirectory with summary_key as summary key.
self.put_current(text, summary=self.getOption('summary'))
def main(*args):
"""
Process command line arguments and invoke bot.
If args is an empty list, sys.argv is used.
@param args: command line arguments
@type args: list of unicode
"""
options = {}
# Process global arguments to determine desired site
local_args = pywikibot.handle_args(args)
# This factory is responsible for processing command line arguments
# that are also used by other scripts and that determine on which pages
# to work on.
genFactory = pagegenerators.GeneratorFactory()
# Parse command line arguments
for arg in local_args:
# Catch the pagegenerators options
if genFactory.handleArg(arg):
continue # nothing to do here
# Now pick up your own options
arg, sep, value = arg.partition(':')
option = arg[1:]
if option in ('summary', 'text'):
if not value:
pywikibot.input('Please enter a value for ' + arg)
options[option] = value
# take the remaining options as booleans.
# You will get a hint if they aren't pre-defined in your bot class
else:
options[option] = True
# The preloading option is responsible for downloading multiple
# pages from the wiki simultaneously.
gen = genFactory.getCombinedGenerator(preload=True)
if gen:
# pass generator and private options to the bot
bot = BasicBot(gen, **options)
bot.run() # guess what it does
return True
else:
pywikibot.bot.suggest_help(missing_generator=True)
return False
if __name__ == '__main__':
main()
| jayvdb/pywikibot-core | scripts/basic.py | Python | mit | 6,777 |
#[PROTEXCAT]
#\License: ALL RIGHTS RESERVED
"""
@file cpuusage.py
"""
##
# @addtogroup pnp pnp
# @brief This is pnp component
# @{
# @addtogroup cpuusage cpuusage
# @brief This is cpuusage module
# @{
##
import os
from oeqa.oetest import oeRuntimeTest
from oeqa.utils.helper import collect_pnp_log
class CPUUsageTest(oeRuntimeTest):
"""CPU consumption for system idle
@class CPUUsageTest
"""
def _reboot(self):
"""reboot device for clean env
@fn _reboot
@param self
@return
"""
(status, output) = self.target.run("reboot")
time.sleep(120)
self.assertEqual(status, 0, output)
def test_cpuusage(self):
"""Measure system idle CPU usage
@fn test_cpuusage
@param self
@return
"""
# self._reboot()
filename = os.path.basename(__file__)
casename = os.path.splitext(filename)[0]
(status, output) = self.target.run(
"top -b -d 10 -n 12 >/tmp/top.log")
(status, output) = self.target.run(
"cat /tmp/top.log | grep -i 'CPU' | grep 'id*' | "
"tail -10 | awk '{print $8}' | "
"awk -F '%' '{sum+=$1} END {print sum/NR}'")
cpu_idle = float(output)
cpu_idle = float("{0:.2f}".format(cpu_idle))
cpu_used = str(100 - cpu_idle) + "%"
collect_pnp_log(casename, casename, cpu_used)
print "\n%s:%s\n" % (casename, cpu_used)
##
# TESTPOINT: #1, test_cpuusage
#
self.assertEqual(status, 0, cpu_used)
(status, output) = self.target.run(
"cat /tmp/top.log")
logname = casename + "-topinfo"
collect_pnp_log(casename, logname, output)
##
# @}
# @}
##
| ostroproject/meta-iotqa | lib/oeqa/runtime/pnp/cpuusage.py | Python | mit | 1,757 |
#-------------------------------------------------------------------------
# Copyright (c) Microsoft. All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
#--------------------------------------------------------------------------
__author__ = 'Microsoft Corp. <[email protected]>'
__version__ = '0.20.6'
_USER_AGENT_STRING = 'pyazure/' + __version__
#-----------------------------------------------------------------------------
# Constants for Azure app environment settings.
AZURE_MANAGEMENT_CERTFILE = 'AZURE_MANAGEMENT_CERTFILE'
AZURE_MANAGEMENT_SUBSCRIPTIONID = 'AZURE_MANAGEMENT_SUBSCRIPTIONID'
# Live ServiceClient URLs
MANAGEMENT_HOST = 'management.core.windows.net'
# Default timeout for http requests (in secs)
DEFAULT_HTTP_TIMEOUT = 65
# x-ms-version for service management.
X_MS_VERSION = '2014-10-01'
| SUSE/azure-sdk-for-python | azure-servicemanagement-legacy/azure/servicemanagement/constants.py | Python | mit | 1,337 |
"""
TinyURL.com shortener implementation
No config params needed
"""
from .base import BaseShortener
from ..exceptions import ShorteningErrorException
class Tinyurl(BaseShortener):
api_url = 'http://tinyurl.com/api-create.php'
def short(self, url):
response = self._get(self.api_url, params=dict(url=url))
if response.ok:
return response.text
raise ShorteningErrorException('There was an error shortening this '
'url - {0}'.format(response.content))
| RuiNascimento/krepo | script.areswizard/pyshorteners/shorteners/tinyurl.py | Python | gpl-2.0 | 536 |
# -*- coding: utf-8 -*-
#
# This file is part of EUDAT B2Share.
# Copyright (C) 2016 CERN.
#
# B2Share is free software; you can redistribute it and/or
# modify it under the terms of the GNU General Public License as
# published by the Free Software Foundation; either version 2 of the
# License, or (at your option) any later version.
#
# B2Share is distributed in the hope that it will be useful, but
# WITHOUT ANY WARRANTY; without even the implied warranty of
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
# General Public License for more details.
#
# You should have received a copy of the GNU General Public License
# along with B2Share; if not, write to the Free Software Foundation, Inc.,
# 59 Temple Place, Suite 330, Boston, MA 02111-1307, USA.
#
# In applying this license, CERN does not
# waive the privileges and immunities granted to it by virtue of its status
# as an Intergovernmental Organization or submit itself to any jurisdiction.
"""Test B2Share record module utils."""
from b2share_unit_tests.helpers import create_deposit
from b2share.modules.records.utils import is_publication, is_deposit
from b2share_unit_tests.helpers import create_user
def test_records_type_helpers(app, test_records_data):
"""Test record util functions retrieving the record type."""
with app.app_context():
creator = create_user('creator')
deposit = create_deposit(test_records_data[0], creator)
deposit.submit()
deposit.publish()
_, record = deposit.fetch_published()
assert is_deposit(deposit.model)
assert not is_deposit(record.model)
assert is_publication(record.model)
assert not is_publication(deposit.model)
| EUDAT-B2SHARE/b2share | tests/b2share_unit_tests/records/test_records_utils.py | Python | gpl-2.0 | 1,720 |
# Test script to compare transform-matrix vlaues in Blender
# Load in blender text editor, select one or more empties and hit [ALT][P]
# ***** BEGIN GPL LICENSE BLOCK *****
#
# This program is free software; you can redistribute it and/or
# modify it under the terms of the GNU General Public License
# as published by the Free Software Foundation; either version 2
# of the License, or (at your option) any later version.
#
# This program is distributed in the hope that it will be useful,
# but WITHOUT ANY WARRANTY; without even the implied warranty of
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
# GNU General Public License for more details.
#
# You should have received a copy of the GNU General Public License
# along with this program; if not, write to the Free Software Foundation,
# Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
#
# ***** END GPL LICENCE BLOCK *****
import Blender
from Blender import *
from Blender.Window import *
from Blender.Object import *
from Blender.Mathutils import *
import math
import struct, string
from types import *
objects = Blender.Object.GetSelected()
for object in objects:
if object.getType()=="Empty":
origin = object.getLocation('worldspace')
matrix = object.getMatrix('worldspace')
print "Name", object.name
print " Origin:", origin[0], " ",origin[1]," ",origin[2]
print " Matrix:"
print "", matrix[0][0], " ",matrix[0][1]," ",matrix[0][2]
print "", matrix[1][0], " ",matrix[1][1]," ",matrix[1][2]
print "", matrix[2][0], " ",matrix[2][1]," ",matrix[2][2]
| chrisglass/ufoai | src/tools/blender/test_matrix.py | Python | gpl-2.0 | 1,565 |
# -*- coding: utf-8 -*-
#
# hl_api_simulation.py
#
# This file is part of NEST.
#
# Copyright (C) 2004 The NEST Initiative
#
# NEST is free software: you can redistribute it and/or modify
# it under the terms of the GNU General Public License as published by
# the Free Software Foundation, either version 2 of the License, or
# (at your option) any later version.
#
# NEST is distributed in the hope that it will be useful,
# but WITHOUT ANY WARRANTY; without even the implied warranty of
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
# GNU General Public License for more details.
#
# You should have received a copy of the GNU General Public License
# along with NEST. If not, see <http://www.gnu.org/licenses/>.
"""
Functions for simulation control
"""
from contextlib import contextmanager
from ..ll_api import *
from .hl_api_helper import *
__all__ = [
'Cleanup',
'DisableStructuralPlasticity',
'EnableStructuralPlasticity',
'GetKernelStatus',
'Install',
'Prepare',
'ResetKernel',
'Run',
'RunManager',
'SetKernelStatus',
'Simulate',
]
@check_stack
def Simulate(t):
"""Simulate the network for `t` milliseconds.
Parameters
----------
t : float
Time to simulate in ms
See Also
--------
RunManager
"""
sps(float(t))
sr('ms Simulate')
@check_stack
def Run(t):
"""Simulate the network for `t` milliseconds.
Parameters
----------
t : float
Time to simulate in ms
Notes
------
Call between `Prepare` and `Cleanup` calls, or within a
``with RunManager`` clause.
Simulate(t): t' = t/m; Prepare(); for _ in range(m): Run(t'); Cleanup()
`Prepare` must be called before `Run` to calibrate the system, and
`Cleanup` must be called after `Run` to close files, cleanup handles, and
so on. After `Cleanup`, `Prepare` can and must be called before more `Run`
calls. Any calls to `SetStatus` between `Prepare` and `Cleanup` have
undefined behaviour.
See Also
--------
Prepare, Cleanup, RunManager, Simulate
"""
sps(float(t))
sr('ms Run')
@check_stack
def Prepare():
"""Calibrate the system before a `Run` call. Not needed for `Simulate`.
Call before the first `Run` call, or before calling `Run` after changing
the system, calling `SetStatus` or `Cleanup`.
See Also
--------
Run, Cleanup
"""
sr('Prepare')
@check_stack
def Cleanup():
"""Cleans up resources after a `Run` call. Not needed for `Simulate`.
Closes state for a series of runs, such as flushing and closing files.
A `Prepare` is needed after a `Cleanup` before any more calls to `Run`.
See Also
--------
Run, Prepare
"""
sr('Cleanup')
@contextmanager
def RunManager():
"""ContextManager for `Run`
Calls `Prepare` before a series of `Run` calls, and calls `Cleanup` at end.
E.g.:
::
with RunManager():
for i in range(10):
Run()
See Also
--------
Prepare, Run, Cleanup, Simulate
"""
Prepare()
try:
yield
finally:
Cleanup()
@check_stack
def ResetKernel():
"""Reset the simulation kernel.
This will destroy the network as well as all custom models created with
:py:func:`.CopyModel`. Calling this function is equivalent to restarting NEST.
In particular,
* all network nodes
* all connections
* all user-defined neuron and synapse models
are deleted, and
* time
* random generators
are reset. The only exception is that dynamically loaded modules are not
unloaded. This may change in a future version of NEST.
"""
sr('ResetKernel')
@check_stack
def SetKernelStatus(params):
"""Set parameters for the simulation kernel.
Parameters
----------
params : dict
Dictionary of parameters to set.
Params dictionary
Some of the keywords in the kernel status dictionary are internally
calculated, and cannot be defined by the user. These are flagged as
`read only` in the parameter list. Use GetKernelStatus to access their
assigned values.
Time and resolution
Parameters
----------
resolution : float
The resolution of the simulation (in ms)
time : float
The current simulation time (in ms)
to_do : int, read only
The number of steps yet to be simulated
max_delay : float
The maximum delay in the network
min_delay : float
The minimum delay in the network
ms_per_tic : float
The number of milliseconds per tic
tics_per_ms : float
The number of tics per millisecond
tics_per_step : int
The number of tics per simulation time step
T_max : float, read only
The largest representable time value
T_min : float, read only
The smallest representable time value
Parallel processing
Parameters
----------
total_num_virtual_procs : int
The total number of virtual processes
local_num_threads : int
The local number of threads
num_processes : int, read only
The number of MPI processes
off_grid_spiking : bool
Whether to transmit precise spike times in MPI communication
grng_seed : int
Seed for global random number generator used synchronously by all
virtual processes to create, e.g., fixed fan-out connections.
rng_seeds : array
Seeds for the per-virtual-process random number generators used for
most purposes. Array with one integer per virtual process, all must
be unique and differ from grng_seed.
MPI buffers
Parameters
----------
adaptive_spike_buffers : bool
Whether MPI buffers for communication of spikes resize on the fly
adaptive_target_buffers : bool
Whether MPI buffers for communication of connections resize on the fly
buffer_size_secondary_events : int, read only
Size of MPI buffers for communicating secondary events (in bytes, per
MPI rank, for developers)
buffer_size_spike_data : int
Total size of MPI buffer for communication of spikes
buffer_size_target_data : int
Total size of MPI buffer for communication of connections
growth_factor_buffer_spike_data : float
If MPI buffers for communication of spikes resize on the fly, grow
them by this factor each round
growth_factor_buffer_target_data : float
If MPI buffers for communication of connections resize on the fly, grow
them by this factor each round
max_buffer_size_spike_data : int
Maximal size of MPI buffers for communication of spikes.
max_buffer_size_target_data : int
Maximal size of MPI buffers for communication of connections
Waveform relaxation method (wfr)
Parameters
----------
use_wfr : bool
Whether to use waveform relaxation method
wfr_comm_interval : float
Desired waveform relaxation communication interval
wfr_tol : float
Convergence tolerance of waveform relaxation method
wfr_max_iterations : int
Maximal number of iterations used for waveform relaxation
wfr_interpolation_order : int
Interpolation order of polynomial used in wfr iterations
Synapses
Parameters
----------
max_num_syn_models : int, read only
Maximal number of synapse models supported
sort_connections_by_source : bool
Whether to sort connections by their source; increases construction
time of presynaptic data structures, decreases simulation time if the
average number of outgoing connections per neuron is smaller than the
total number of threads
structural_plasticity_synapses : dict
Defines all synapses which are plastic for the structural plasticity
algorithm. Each entry in the dictionary is composed of a synapse model,
the pre synaptic element and the postsynaptic element
structural_plasticity_update_interval : int
Defines the time interval in ms at which the structural plasticity
manager will make changes in the structure of the network (creation
and deletion of plastic synapses)
Output
Returns
-------
data_path : str
A path, where all data is written to (default is the current
directory)
data_prefix : str
A common prefix for all data files
overwrite_files : bool
Whether to overwrite existing data files
print_time : bool
Whether to print progress information during the simulation
network_size : int, read only
The number of nodes in the network
num_connections : int, read only, local only
The number of connections in the network
local_spike_counter : int, read only
Number of spikes fired by neurons on a given MPI rank since NEST was
started or the last ResetKernel. Only spikes from "normal" neurons
(neuron models with proxies) are counted, not spikes generated by
devices such as poisson_generator.
Miscellaneous
Other Parameters
----------------
dict_miss_is_error : bool
Whether missed dictionary entries are treated as errors
keep_source_table : bool
Whether to keep source table after connection setup is complete
See Also
--------
GetKernelStatus
"""
sps(params)
sr('SetKernelStatus')
@check_stack
def GetKernelStatus(keys=None):
"""Obtain parameters of the simulation kernel.
Parameters
----------
keys : str or list, optional
Single parameter name or `list` of parameter names
Returns
-------
dict:
Parameter dictionary, if called without argument
type:
Single parameter value, if called with single parameter name
list:
List of parameter values, if called with list of parameter names
Raises
------
TypeError
If `keys` are of the wrong type.
Notes
-----
See SetKernelStatus for documentation on each parameter key.
See Also
--------
SetKernelStatus
"""
sr('GetKernelStatus')
status_root = spp()
if keys is None:
return status_root
elif is_literal(keys):
return status_root[keys]
elif is_iterable(keys):
return tuple(status_root[k] for k in keys)
else:
raise TypeError("keys should be either a string or an iterable")
@check_stack
def Install(module_name):
"""Load a dynamically linked NEST module.
Parameters
----------
module_name : str
Name of the dynamically linked module
Returns
-------
handle
NEST module identifier, required for unloading
Notes
-----
Dynamically linked modules are searched in the NEST library
directory (``<prefix>/lib/nest``) and in ``LD_LIBRARY_PATH`` (on
Linux) or ``DYLD_LIBRARY_PATH`` (on OSX).
**Example**
::
nest.Install("mymodule")
"""
return sr("(%s) Install" % module_name)
@check_stack
def EnableStructuralPlasticity():
"""Enable structural plasticity for the network simulation
See Also
--------
DisableStructuralPlasticity
"""
sr('EnableStructuralPlasticity')
@check_stack
def DisableStructuralPlasticity():
"""Disable structural plasticity for the network simulation
See Also
--------
EnableStructuralPlasticity
"""
sr('DisableStructuralPlasticity')
| SepehrMN/nest-simulator | pynest/nest/lib/hl_api_simulation.py | Python | gpl-2.0 | 11,564 |
#
# Copyright (C) 2006, 2007, One Laptop Per Child
#
# This program is free software; you can redistribute it and/or modify
# it under the terms of the GNU General Public License as published by
# the Free Software Foundation; either version 2 of the License, or
# (at your option) any later version.
#
# This program is distributed in the hope that it will be useful,
# but WITHOUT ANY WARRANTY; without even the implied warranty of
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
# GNU General Public License for more details.
#
# You should have received a copy of the GNU General Public License
# along with this program; if not, write to the Free Software
# Foundation, Inc., 675 Mass Ave, Cambridge, MA 02139, USA.
#
import json
import sha
from gi.repository import GObject
import base64
class Model(GObject.GObject):
''' The model of web-activity which uses json to serialize its data
to a file and deserealize from it.
'''
__gsignals__ = {
'add_link': (GObject.SignalFlags.RUN_FIRST,
None, ([int])),
}
def __init__(self):
GObject.GObject.__init__(self)
self.data = {}
self.data['shared_links'] = []
self.data['deleted'] = []
def add_link(self, url, title, thumb, owner, color, timestamp):
index = len(self.data['shared_links'])
for item in self.data['shared_links']:
if timestamp <= item['timestamp']:
index = self.data['shared_links'].index(item)
break
info = {'hash': sha.new(str(url)).hexdigest(), 'url': str(url),
'title': str(title), 'thumb': base64.b64encode(thumb),
'owner': str(owner), 'color': str(color),
'timestamp': float(timestamp)}
self.data['shared_links'].insert(index, info)
self.emit('add_link', index)
def remove_link(self, hash):
for link in self.data['shared_links']:
if link['hash'] == hash:
self.data['deleted'].append(link['hash'])
self.data['shared_links'].remove(link)
break
def change_link_notes(self, hash, notes):
for link in self.data['shared_links']:
if link['hash'] == hash:
link['notes'] = notes
def serialize(self):
return json.dumps(self.data)
def deserialize(self, data):
self.data = json.loads(data)
self.data.setdefault('shared_links', [])
self.data.setdefault('deleted', [])
def get_links_ids(self):
ids = []
for link in self.data['shared_links']:
ids.append(link['hash'])
ids.extend(self.data['deleted'])
ids.append('')
return ids
| iamutkarshtiwari/Browse-fiddleFeature | model.py | Python | gpl-2.0 | 2,775 |
# -*- coding: utf-8 -*-
'''
Created on 21/01/2012
Copyright (c) 2010-2012 Shai Bentin.
All rights reserved. Unpublished -- rights reserved
Use of a copyright notice is precautionary only, and does
not imply publication or disclosure.
Licensed under Eclipse Public License, Version 1.0
Initial Developer: Shai Bentin.
@author: shai
'''
from APLoader import APLoader
class APEpgLoader(APLoader):
'''
classdocs
'''
EPG_URI = "v{{api_version}}/accounts/{{account_id}}/broadcasters/{{broadcaster_id}}/vod_items/{{item_id}}/epg"
def __init__(self, settings, itemId = ''):
'''
Constructor
'''
super(APEpgLoader, self).__init__(settings) # call the parent constructor with the settings object
self.queryUrl = self.URL + self.EPG_URI
self.queryUrl = self.queryUrl.replace("{{api_version}}", "1" + "2")
self.queryUrl = self.queryUrl.replace("{{account_id}}", self.accountId)
self.queryUrl = self.queryUrl.replace("{{broadcaster_id}}", self.broadcasterId)
self.queryUrl = self.queryUrl.replace("{{item_id}}", itemId);
self.queryUrl = self.prepareQueryURL(self.queryUrl, None); | guymakam/Kodi-Israel | plugin.video.reshet.video/resources/appCaster/APEpgLoader.py | Python | gpl-2.0 | 1,230 |
# $Id: 200_register.py 369517 2012-07-01 17:28:57Z file $
#
from inc_cfg import *
# Basic registration
test_param = TestParam(
"Basic registration",
[
InstanceParam( "client",
"--null-audio"+
" --id=\"<sip:[email protected]>\""+
" --registrar=sip:sip.pjsip.org" +
" --username=test1" +
" --password=test1" +
" --realm=*",
uri="sip:[email protected]",
have_reg=True),
]
)
| fluentstream/asterisk-p2p | res/pjproject/tests/pjsua/scripts-run/200_register.py | Python | gpl-2.0 | 426 |
# python version 1.0 DO NOT EDIT
#
# Generated by smidump version 0.4.8:
#
# smidump -f python PDU2-MIB
FILENAME = "pdu2_mib.mib"
MIB = {
"moduleName" : "PDU2-MIB",
"PDU2-MIB" : {
"nodetype" : "module",
"language" : "SMIv2",
"organization" :
"""Raritan""",
"contact" :
"""
Author: Raritan Americas, Inc.
postal: Raritan Americas, Inc.
400 Cottontail Lane
Somerset, NJ 08873
email: [email protected]
phone: +1 732 764 8886""",
"description" :
"""This MIB describes the SNMP functions of the Dominion PX G2
Power Distribution Unit by Raritan Computer.""",
"revisions" : (
{
"date" : "2016-02-09 00:00",
"description" :
"""PX2 release 3.2.20:
1) Added the following:
NetworkInterfaceTypeEnumeration,
networkInterfaceType to unitConfigurationTable
2) Added AddressSourceEnumeration
3) Added activeDNS ServerCount to unitConfigurationTable
4) Added activeDNSServerTable""",
},
{
"date" : "2015-10-26 00:00",
"description" :
"""PX2 release 3.2.10:
1) Added to trapInformation:
phoneNumber
Added the following traps:
smsMessageTransmissionFailure trap""",
},
{
"date" : "2015-09-30 00:00",
"description" :
"""PX2 release 3.2.1:
- Support for PMC/BCM2
- Line and node information for component poles
1) SensorTypeEnumeration: Added
reactivePower(29)
displacementPowerFactor(35)
2) SensorUnitsEnumeration: Added
var(23)
3) ProductTypeEnumeration: Added
powerMeter(3)
4) Added the following enumerations:
PanelLayoutEnumeration
PanelNumberingEnumeration
CircuitTypeEnumeration
PhaseEnumeration
LineEnumeration
PowerMeterTypeEnumeration
5) Added the following tables:
inletPoleConfigurationTable
overCurrentProtectorPoleConfigurationTable
outletPoleConfigurationTable
transferSwitchPoleConfigurationTable
powerMeterConfigurationTable
circuitConfigurationTable
circuitPoleConfigurationTable
circuitSensorConfigurationTable
circuitPoleSensorConfigurationTable
circuitSensorLogTable
circuitPoleSensorLogTable
circuitSensorMeasurementsTable
circuitPoleSensorMeasurementsTable
circuitSensorControlTable
6) unitConfigurationTable: Added
circuitCount
7) inletDeviceCapabilities: Added
reactivePower(28)
8) inletPoleCapabilities: Added
phaseAngle(23)
reactivePower(28)
displacementPowerFactor(34)
9) overCurrentProtectorConfigurationTable: Added
overCurrentProtectorPoleCount
10) transferSwitchConfigurationTable: Added
transferSwitchPoleCount
11) Added the following to trapInformation:
circuitNumber
circuitPoleNumber
12) Added the following traps:
circuitSensorStateChange
circuitPoleSensorStateChange
circuitAdded
circuitDeleted
circuitModified
circuitSensorReset
powerMeterAdded
powerMeterDeleted
powerMeterModified
13) Revised the DESCRIPTION clauses of:
pduCount
pduId
inletPoleCount""",
},
{
"date" : "2015-02-18 00:00",
"description" :
"""PX2 release 3.1.0:
- Support absolute humidity sensors
1) SensorStateEnumeration: Added
nonRedundant(30)
2) SensorTypeEnumeration: Added
absoluteHumidity(28)
3) SensorUnitsEnumeration: Added
grampercubicmeter(22)
4) Corrected the possible states for the rcmState, operatingState and activeInlet sensors
5) Documented that the unitSensorResetValue object in unitSensorControlTable applies
only to multi-inlet PDUs
6) Deprecated inletRatedVA, inletRatedFrequency
7) inletDeviceCapabilities: Corrected bit values for residualCurrent(25)
and rcmState(26)""",
},
{
"date" : "2014-06-04 00:00",
"description" :
"""PX2 release 3.0.0:
- Support for signed sensor readings
- Support for unit-level power and energy sensors
- Send trap on peripheral device firmware update
- Allow peripheral device auto management to be disabled
- Allow front panel outlet switching to be enabled or disabled
- Support DX-PIR occupancy sensor
1) Changed the SYNTAX from Integer32 to Unsigned32:
measurementsUnitSensorValue
2) Added signed value, range and threshold columns to:
unitSensorConfigurationTable
unitSensorMeasurementsTable
pduSensorStateChange
inletSensorConfigurationTable
inletSensorMeasurementsTable
inletSensorLogTable
inletSensorStateChange
inletPoleSensorConfigurationTable
inletPoleSensorMeasurementsTable
inletPoleSensorLogTable
inletPoleSensorStateChange
overCurrentProtectorSensorConfigurationTable
overCurrentProtectorSensorMeasurementsTable
overCurrentProtectorSensorLogTable
overCurrentProtectorSensorStateChange
outletSensorConfigurationTable
outletSensorMeasurementsTable
outletSensorLogTable
outletSensorStateChange
outletPoleSensorConfigurationTable
outletPoleSensorMeasurementsTable
outletPoleSensorLogTable
outletPoleSensorStateChange
3) Added unsigned value, range and threshold columns to:
unitSensorLogTable
transferSwitchSensorConfigurationTable
transferSwitchSensorMeasurementsTable
transferSwitchSensorLogTable
transferSwitchSensorStateChange
4) Added UnitSensorControlTable
5) Added unitSensorReset and unitSensorStateChange traps
6) Deprecated pduSensorStateChange trap
7) Added to unitConfigurationTable:
peripheralDevicesAutoManagement
frontPanelOutletSwitching
frontPanelRCMSelfTest
frontPanelActuatorControl
8) Added to externalSensorConfigurationTable:
externalSensorAlarmedToNormalDelay
9) Deprecated:
wireCount in unitConfiguraionTable
tables for wireSensors
traps for wireSensors
10) SensorStateEnumeration: Added
fail(14)
11) ProductTypeEnumeration: Renamed
rackSts(2) to transferSwitch
12) Added:
PeripheralDeviceFirmwareUpdateStateEnumeration
peripheralDeviceFirmwareUpdateState
peripheralDeviceFirmwareUpdate trap
13) Added userName to the following traps:
bulkConfigurationSaved
bulkConfigurationCopied
lhxSupportChanged
deviceSettingsSaved
deviceSettingsRestored""",
},
{
"date" : "2014-01-09 00:00",
"description" :
"""PX2 release 2.6.0:
- Support for PX3TS transfer switches
1) SensorTypeEnumeration:
Removed:
scrOpenStatus
scrShortStatus
Added:
i1SmpsStatus(46)
i2SmpsStatus(47)
switchStatus(48)
2) SensorStateEnumeration:
Removed:
marginal
fail
Added:
i1OpenFault(22)
i1ShortFault(23)
i2OpenFault(24)
i2ShortFault(25)
fault(26)
warning(27)
critical(28)
3) unitDeviceCapabilities: Added
i1SmpsStatus(45)
i2SmpsStatus(46)
4) transferSwitchCapabilities: Added
switchStatus(47)
5) Added transferSwitchConfiguration table
6) unitSensorLogTable:
Removed:
logUnitSensorAvgValue
logUnitSensorMaxValue
logUnitSensorMinValue
Added:
logUnitSensorSignedAvgValue
logUnitSensorSignedMaxValue
logUnitSensorSignedMinValue""",
},
{
"date" : "2014-01-07 00:00",
"description" :
"""PX2 release 2.5.30:
- Accumulating sensors (energy counters) can be reset
- Sensor accuray and tolerance variables are deprecated
1) Added peripheralDevicePackagePosition and peripheralDevicePackageState
2) Added radiusError trap
3) Added serverReachabilityError trap
4) Deprecated the following:
unitSensorConfigurationTable/unitSensorAccuracy
unitSensorConfigurationTable/unitSensorTolerance
inletSensorConfigurationTable/inletSensorAccuracy
inletSensorConfigurationTable/inletSensorTolerance
inletPoleSensorConfigurationTable/inletPoleSensorAccuracy
inletPoleSensorConfigurationTable/inletPoleSensorTolerance
outletSensorConfigurationTable/outetSensorAccuracy
outletSensorConfigurationTable/outletSensorTolerance
outletPoleSensorConfigurationTable/outetPoleSensorAccuracy
outletPoleSensorConfigurationTable/outletPoleSensorTolerance
overCurrentProtectorSensorConfigurationTable/overCurrentProtectorSensorAccuracy
overCurrentProtectorSensorConfigurationTable/overCurrentProtectorSensorTolerance
externalSensorConfigurationTable/externalSensorAccuracy
externalSensorConfigurationTable/externalSensorTolerance
wireSensorConfigurationTable/wireSensorAccuracy
wireSensorConfigurationTable/wireSensorTolerance
transferSwitchSensorConfigurationTable/transferSwitchSensorAccuracy
transferSwitchSensorConfigurationTable/transferSwitchSensorTolerance
5) Added inletSensorReset and outletSensorReset traps
6) Added inletSensorControl and inletSensorControlTable
7) Added outletSensorControl and outletSensorControlTable
8) Added unknownPeripheralDeviceAttached trap""",
},
{
"date" : "2013-11-21 00:00",
"description" :
"""PX2 release 2.5.20:
- Support for residual current monitors
- Support for USB cascading with one IP address
- Support for line-neutral voltage sensors
1) SensorTypeEnumeration: Added
rmsVoltageLN(25)
residualCurrent(26)
rcmState(27)
2) SensorStateEnumeration: Added
warning(27)
critical(28)
selfTest(29)
3) inletDeviceCapabilities: Added
residualCurrent(26)
rcmState(27)
4) Added rmsVoltageLN(24) to inletPoleCapabilities
5) Added inletRCMResidualOperatingCurrent to inletConfigurationTable
6) Added rcmControl under control
7) Added rcmSelfTestTable under rcmControl
8) Added DeviceCascadeTypeEnumeration
9) Added deviceCascadeType, deviceCascadePosition to unitConfigurationTable
10) Added agentInetPortNumber under trapInformation
11) Added agentInetPortNumber as a varbind to all traps
12) Added peripheralDevicePackageTable containing information on peripheral
device packages""",
},
{
"date" : "2013-09-18 00:00",
"description" :
"""
1) Added serverConnectivityUnrecoverable trap""",
},
{
"date" : "2013-08-01 00:00",
"description" :
"""
1) Add RCBO OCP types""",
},
{
"date" : "2013-07-10 00:00",
"description" :
"""
1) Added externalSensorTypeDefaultThresholdsTable """,
},
{
"date" : "2013-07-02 00:00",
"description" :
"""
1) Added relayBehaviorOnPowerLoss to unitConfigurationTable""",
},
{
"date" : "2013-05-21 00:00",
"description" :
"""
1) Added inletEnableState to inletConfigurationTable
2) Added traps: inletEnabled and inletDisabled""",
},
{
"date" : "2013-04-26 00:00",
"description" :
"""
1) Added traps: webcamInserted and webcamRemoved
2) Added trapInformation parameters: webcamModel,webcamConnectionPort""",
},
{
"date" : "2013-03-27 00:00",
"description" :
"""
1) Changed outletSource to outletPowerSource in outletConfigurationTable
2) Changed transferSwitchSource1 and transferSwitchSource2 to
transferSwitchPowerSource1 and transferSwitchPowerSource2 in transferSwitchConfigurationTable
3) Changed overCurrentProtectorSource to overCurrentProtectorPowerSource in overCurrentProtectorConfigurationTable
4) Changed wireSource to wirePowerSource in wireConfigurationTable""",
},
{
"date" : "2013-03-25 10:00",
"description" :
"""
1) Added comments showing the possible states for each sensor type. """,
},
{
"date" : "2013-03-25 00:00",
"description" :
"""
1) Added outletSource to outletConfigurationTable
2) Added transferSwitchSource1 and transferSwitchSource2 to transferSwitchConfigurationTable
3) Added overCurrentProtectorSource to overCurrentProtectorConfigurationTable
4) Added wireSource to wireConfigurationTable""",
},
{
"date" : "2013-03-18 00:00",
"description" :
""" 1) Added meteringControllerCount to the unitConfigurationTable
2) Added meteringController to BoardTypeEnumeration""",
},
{
"date" : "2013-02-25 00:00",
"description" :
"""
1) Added ProductTypeEnumeration
2) Added productType to unitConfigurationTable""",
},
{
"date" : "2013-02-04 00:00",
"description" :
""" 1) Added TransferSwitchTransferReasonEnumeration
2) Added transferSwitchLastTransferReason to transferSwitchControlTable
3) Added transferSwitchLastTransferReason to transferSwitchSensorStateChange trap""",
},
{
"date" : "2013-01-24 00:00",
"description" :
"""Added required sensor types and units""",
},
{
"date" : "2012-11-20 00:00",
"description" :
"""1) Added externalSensorIsActuator and externalSensorPosition to the externalSensorConfigurationTable
2) Added actuatorControlTable""",
},
{
"date" : "2012-11-15 00:00",
"description" :
"""1) Removed transferSwitchOutputCapabilities from transferSwitchConfigurationTable
2) Removed the following tables:
transferSwitchOutputSensorConfigurationTable
transferSwitchOutputSensorLogTable
transferSwitchOutputSensorMeasurementsTable
3) Removed transferSwitchOutputSensorStateChange trap
4) Added transferSwitchControlTable
5) Removed the following entries from SensorTypeEnumeration:
overTemperatureFault
fans
internalFault
inletPhaseDeviationFault
overloadFault
6) Added the following entries to SensorTypeEnumeration:
overloadStatus
overheatStatus
scrOpenStatus
scrShortStatus
fanStatus
inletPhaseSyncAngle
inletPhaseSync
7) Added the following entries to SensorStateEnumeration:
inSync,
outOfSync
8) Renamed transferSwitchNoTransferIfPhaseDeviationFault to
transferSwitchAutoReTransferRequiresPhaseSync""",
},
{
"date" : "2012-10-05 00:00",
"description" :
"""1) Modified the DESCRIPTION of the following.
outletSwitchControlTable
transferSwitchOutputSensorMeasurementsEntry
overCurrentProtectorSensorMeasurementsEntry
outletPoleSensorMeasurementsEntry
transferSwitchOutputSensorLogEntry
transferSwitchOutputSensorLogTable
wireSensorLogEntry
externalSensorNumber
controllerConfigurationEntry
SensorUnitsEnumeration
measurementsGroup
logGroup""",
},
{
"date" : "2012-10-04 00:00",
"description" :
"""1) In the transferSwitchConfigurationTable,
replaced transferSwitchFrequencyDeviation with
transferSwitchLowerMarginalFrequency and transferSwitchUpperMarginalFrequency""",
},
{
"date" : "2012-09-28 00:00",
"description" :
"""1) Modified the DESCRIPTION of the following.
bulkConfigurationCopied, userModified, userSessionTimeout""",
},
{
"date" : "2012-09-21 00:00",
"description" :
"""1) Added the following traps:
deviceSettingsSaved, deviceSettingsRestored""",
},
{
"date" : "2012-09-20 00:00",
"description" :
"""1) Added the following objects to the transferSwitchConfigurationTable:
transferSwitchInternalFaultType
2) Added transferSwitchInternalFaultType to transferSwitchSensorStateChange trap
3) Added marginal to SensorStateEnumeration""",
},
{
"date" : "2012-09-17 00:00",
"description" :
"""Deprecated the following objects from the unitConfigurationTable
pxInetAddressType,
pxInetIPAddress,
pxInetNetmask,
pxInetGateway """,
},
{
"date" : "2012-09-04 00:00",
"description" :
"""Support for transfer Switch objects and sensors.
1. Added transferSwitchCount to unitConfigurationTable
2. Added the following tables:
transferSwitchConfigurationTable,
transferSwitchSensorConfigurationTable,
transferSwitchSensorLogTable,
transferSwitchSensorMeasurementsTable
transferSwitchOutputSensorConfigurationTable,
transferSwitchOutputSensorLogTable,
transferSwitchOutputSensorMeasurementsTable
3. Added
transferSwitchSensorStateChange trap
transferSwitchOutputSensorStateChange trap""",
},
{
"date" : "2012-06-22 00:00",
"description" :
"""
1. Added surgeProtectorStatus to SensorTypeEnumeration
2. Added surgeProtectorStatus to inletDeviceCapabilities""",
},
{
"date" : "2012-06-18 00:00",
"description" :
"""Added a comment before the section listing the traps.
The comment notes that the pxInetIPAddressType and
pxInetIPAddress fields are not used for IPv6 traps""",
},
{
"date" : "2012-06-06 00:00",
"description" :
"""Support for wire objects and sensors.
1. Added wireCount to unitConfigurationTable
2. Added the following tables:
wireConfigurationTable,
wireSensorConfigurationTable,
wireSensorLogTable,
wireSensorMeasurementsTable
3. Added wireSensorStateChange trap""",
},
{
"date" : "2012-05-25 00:00",
"description" :
"""added userAccepted/DeclinedRestrictedServiceAgreement traps""",
},
{
"date" : "2012-05-15 00:00",
"description" :
"""
1. Added support for NTP servers.
Added the following objects under info
synchronizeWithNTPServer, useDHCPProvidedNTPServer,
firstNTPServerAddressType, firstNTPServerAddress,
secondNTPServerAddressType, secondNTPServerAddress""",
},
{
"date" : "2012-03-26 00:00",
"description" :
"""added lhxSupportChanged trap.""",
},
{
"date" : "2011-12-13 00:00",
"description" :
"""
1. Added usbSlaveConnected, usbSlaveDisonnected traps""",
},
{
"date" : "2011-11-29 00:00",
"description" :
"""
1. Added cascadedDeviceConnected to UnitConfigurationEntryStruct""",
},
{
"date" : "2011-10-25 00:00",
"description" :
"""
1. Added DeviceIdentificationParameterEnumeration
2. Added deviceIdentificationChanged Trap
3. Added sysContact, sysName, sysLocation to all traps""",
},
{
"date" : "2011-06-16 00:00",
"description" :
"""
1. Changed DESCRIPTION of outletSequencingDelay""",
},
{
"date" : "2011-03-22 00:00",
"description" :
"""
1. Added rfCodeTagConnected, rfCodeTagDisconnected traps
2. Changed MAX-ACCESS for externalOnOffSensorSubtype to read-write""",
},
{
"date" : "2011-02-21 00:00",
"description" :
"""
1. Added rpm(19) to SensorUnitsEnumeration""",
},
{
"date" : "2011-02-14 00:00",
"description" :
"""
1. Changed 5WIRE IEC60309 enumerations from 250V to 208V""",
},
{
"date" : "2011-02-08 00:00",
"description" :
"""
1. Removed OnOffSensorSubtypeEnumeration
2. Changed SYNTAX of externalOnOffSensorSubtype to SensorTypeEnumeration
3. Added binary, contact, fanspeed, none to SensorTypeEnumeration
4. Changed outletPoleCapabilities to be the same as inletPoleCapabilities""",
},
{
"date" : "2011-02-03 00:00",
"description" :
"""
1. Added externalSensorSerialNumber,externalOnOffSensorSubtype, externalSensorChannelNumber
to the externalSensorStateChange trap.""",
},
{
"date" : "2011-01-31 00:00",
"description" :
"""
1. Modifed the DESCRIPTION of the powerControl trap""",
},
{
"date" : "2010-12-15 00:00",
"description" :
"""
1. Added dataLoggingEnableForAllSensors to logConfigurationTable""",
},
{
"date" : "2010-12-13 11:31",
"description" :
"""
1. Added inrushGuardDelay to unitConfigurationTable
2. Added outletSequenceDelay to outletConfigurationTable
3. Deprecated outletSequencingDelay""",
},
{
"date" : "2010-12-13 00:00",
"description" :
"""1. Added externalOnOffSensorSubtype to ExternalSensorConfigurationEntryStruct
2. Added OnOffSensorSubtypeEnumeration
3. Added alarmed to SensorStateEnumeration
4. Removed firmwareFileDiscarded trap
5. Removed securityViolation trap""",
},
{
"date" : "2010-12-07 00:00",
"description" :
"""1. changed DESCRIPTION of measurementPeriod to say that the value is fixed at 1 second.""",
},
{
"date" : "2010-10-07 00:00",
"description" :
"""1. added ocpFUSEPAIR(5) to OverCurrentProtectorTypeEnumeration
2. changed ocpFUSE1POLE(4) to ocpFUSE(4) in OverCurrentProtectorTypeEnumeration""",
},
{
"date" : "2010-10-04 00:00",
"description" :
"""1. added ocpFUSE2POLE(5) to OverCurrentProtectorTypeEnumeration
2. changed ocpFUSE(4) to ocpFUSE1POLE(4) in OverCurrentProtectorTypeEnumeration""",
},
{
"date" : "2010-09-01 00:00",
"description" :
"""1. Removed userName from serverNotReachable and serverReachable traps""",
},
{
"date" : "2010-08-05 00:00",
"description" :
"""1. Added reliabilityDataTableSequenceNumber
2. Changed SYNTAX of reliabilityErrorLogIndex to Integer32(1..2147483647)""",
},
{
"date" : "2010-07-23 00:00",
"description" :
"""1. Moved serverCount to unitConfigurationTable """,
},
{
"date" : "2010-07-22 00:00",
"description" :
"""1. Added support for the Reliability Tables
2. Added new group reliabilityGroup
3. Defined nodes reliability,reliabilityData,reliabilityErrorLog
4. Added reliabilityDataTable & reliabilityErrorLogTable""",
},
{
"date" : "2010-07-21 00:00",
"description" :
"""1. Added plug56PA320 to PlugTypeEnumeration
2. Added plug56P320F to PlugTypeEnumeration""",
},
{
"date" : "2010-07-14 00:00",
"description" :
"""1. Added the following traps:
pingServerEnabled, pingServerDisabled, serverNotReachable, serverReachable
2. Added the serverReachabilityTable """,
},
{
"date" : "2010-07-06 00:00",
"description" :
"""1. Added externalSensorChannelNumber to externalSensorConfigurationTable""",
},
{
"date" : "2010-07-01 00:00",
"description" :
"""1. added outletSwitchingState to outletSwitchControlTable
2. added outletSwitchingTimeStamp to outletSwitchControlTable""",
},
{
"date" : "2010-06-30 00:00",
"description" :
"""1. added switchingOperation to the powerControl trap""",
},
{
"date" : "2010-06-21 00:00",
"description" :
"""1. added support for Load shedding
2. added loadShedding to the unitConfigurationTable.
3. added nonCritical to the outletConfigurationTable
4. added loadSheddingModeEntered & loadSheddingModeExited traps
5. modified description of inletPlug in inletConfigurationTable""",
},
{
"date" : "2010-06-03 00:00",
"description" :
"""1. added plugOTHER to PlugTypeEnumeration
2. added receptacleOTHER to ReceptacleTypeEnumeration
3. added inletPlugDescriptor to inletConfigurationTable
4. added outletReceptacleDescriptor to outletConfigurationTable""",
},
{
"date" : "2010-05-27 00:00",
"description" :
"""1. added INetAddressType and INetAddress to represent IP addresses
2. unitConfigurationTable: deprecated pxIpAddress
3. unitConfigurationTable: added pxInetAddressType,pxInetIPAddress,pxInetNetmask,pxInetGateway
2: added pxInetAddressType,pxInetIPAddress to all traps
3: defined new trap deviceUpdateFailed""",
},
{
"date" : "2010-05-24 00:00",
"description" :
"""Added typeOfSensor to externalSensorStateChange trap""",
},
{
"date" : "2010-04-19 00:00",
"description" :
"""modified the DESCRIPTION of the deviceUpdateCompleted trap""",
},
{
"date" : "2010-04-15 00:00",
"description" :
"""modified the DESCRIPTION of all SensorStateChangeDelay parameters""",
},
{
"date" : "2010-04-08 00:00",
"description" :
"""modified the DESCRIPTION of sensor parameters that do not apply to discrete sensors""",
},
{
"date" : "2010-03-29 00:00",
"description" :
"""added trap ldapError""",
},
{
"date" : "2010-03-25 00:00",
"description" :
"""changed the SYNTAX of the following objects from Integer32 to Unsigned32:
logInletSensorMaxValue,
logInletSensorMinValue,
logInletSensorAvgValue,
logInletPoleSensorMaxValue,
logInletPoleSensorMinValue,
logInletPoleSensorAvgValue,
logOutletSensorMaxValue,
logOutletSensorMinValue,
logOutletSensorAvgValue,
logOutletPoleSensorMaxValue,
logOutlePoletSensorMinValue,
logOutletPoleSensorAvgValue,
logOverCurrentProtectorSensorMaxValue,
logOverCurrentProtectorSensorMinValue,
logOverCurrentProtectorSensorAvgValue,
measurementsInletSensorValue,
measurementsInletPoleSensorValue,
measurementsOutletSensorValue,
measurementsOutletPoleSensorValue,
measurementsOverCurrentProtectorSensorValue""",
},
{
"date" : "2010-03-16 00:00",
"description" :
"""added trap smtpMessageTransmissionFailure""",
},
{
"date" : "2010-03-01 00:00",
"description" :
"""changed externalSensorsZCoordinateUnits to an Enumeration""",
},
{
"date" : "2010-01-29 00:00",
"description" :
"""The first version of the MIB.""",
},
),
"identity node" : "raritan",
},
"imports" : (
{"module" : "SNMPv2-SMI", "name" : "MODULE-IDENTITY"},
{"module" : "SNMPv2-SMI", "name" : "OBJECT-TYPE"},
{"module" : "SNMPv2-SMI", "name" : "NOTIFICATION-TYPE"},
{"module" : "SNMPv2-SMI", "name" : "enterprises"},
{"module" : "SNMPv2-SMI", "name" : "Integer32"},
{"module" : "SNMPv2-SMI", "name" : "Unsigned32"},
{"module" : "SNMPv2-SMI", "name" : "IpAddress"},
{"module" : "SNMPv2-CONF", "name" : "MODULE-COMPLIANCE"},
{"module" : "SNMPv2-CONF", "name" : "OBJECT-GROUP"},
{"module" : "SNMPv2-CONF", "name" : "NOTIFICATION-GROUP"},
{"module" : "SNMPv2-TC", "name" : "TEXTUAL-CONVENTION"},
{"module" : "SNMPv2-TC", "name" : "DisplayString"},
{"module" : "SNMPv2-TC", "name" : "MacAddress"},
{"module" : "SNMPv2-TC", "name" : "TruthValue"},
{"module" : "SNMPv2-TC", "name" : "RowPointer"},
{"module" : "INET-ADDRESS-MIB", "name" : "InetAddressType"},
{"module" : "INET-ADDRESS-MIB", "name" : "InetAddress"},
{"module" : "INET-ADDRESS-MIB", "name" : "InetPortNumber"},
{"module" : "RFC1213-MIB", "name" : "sysContact"},
{"module" : "RFC1213-MIB", "name" : "sysName"},
{"module" : "RFC1213-MIB", "name" : "sysLocation"},
),
"typedefs" : {
"SensorTypeEnumeration" : {
"basetype" : "Enumeration",
"status" : "current",
"rmsCurrent" : {
"nodetype" : "namednumber",
"number" : "1"
},
"peakCurrent" : {
"nodetype" : "namednumber",
"number" : "2"
},
"unbalancedCurrent" : {
"nodetype" : "namednumber",
"number" : "3"
},
"rmsVoltage" : {
"nodetype" : "namednumber",
"number" : "4"
},
"activePower" : {
"nodetype" : "namednumber",
"number" : "5"
},
"apparentPower" : {
"nodetype" : "namednumber",
"number" : "6"
},
"powerFactor" : {
"nodetype" : "namednumber",
"number" : "7"
},
"activeEnergy" : {
"nodetype" : "namednumber",
"number" : "8"
},
"apparentEnergy" : {
"nodetype" : "namednumber",
"number" : "9"
},
"temperature" : {
"nodetype" : "namednumber",
"number" : "10"
},
"humidity" : {
"nodetype" : "namednumber",
"number" : "11"
},
"airFlow" : {
"nodetype" : "namednumber",
"number" : "12"
},
"airPressure" : {
"nodetype" : "namednumber",
"number" : "13"
},
"onOff" : {
"nodetype" : "namednumber",
"number" : "14"
},
"trip" : {
"nodetype" : "namednumber",
"number" : "15"
},
"vibration" : {
"nodetype" : "namednumber",
"number" : "16"
},
"waterDetection" : {
"nodetype" : "namednumber",
"number" : "17"
},
"smokeDetection" : {
"nodetype" : "namednumber",
"number" : "18"
},
"binary" : {
"nodetype" : "namednumber",
"number" : "19"
},
"contact" : {
"nodetype" : "namednumber",
"number" : "20"
},
"fanSpeed" : {
"nodetype" : "namednumber",
"number" : "21"
},
"surgeProtectorStatus" : {
"nodetype" : "namednumber",
"number" : "22"
},
"frequency" : {
"nodetype" : "namednumber",
"number" : "23"
},
"phaseAngle" : {
"nodetype" : "namednumber",
"number" : "24"
},
"rmsVoltageLN" : {
"nodetype" : "namednumber",
"number" : "25"
},
"residualCurrent" : {
"nodetype" : "namednumber",
"number" : "26"
},
"rcmState" : {
"nodetype" : "namednumber",
"number" : "27"
},
"absoluteHumidity" : {
"nodetype" : "namednumber",
"number" : "28"
},
"reactivePower" : {
"nodetype" : "namednumber",
"number" : "29"
},
"other" : {
"nodetype" : "namednumber",
"number" : "30"
},
"none" : {
"nodetype" : "namednumber",
"number" : "31"
},
"powerQuality" : {
"nodetype" : "namednumber",
"number" : "32"
},
"overloadStatus" : {
"nodetype" : "namednumber",
"number" : "33"
},
"overheatStatus" : {
"nodetype" : "namednumber",
"number" : "34"
},
"displacementPowerFactor" : {
"nodetype" : "namednumber",
"number" : "35"
},
"fanStatus" : {
"nodetype" : "namednumber",
"number" : "37"
},
"inletPhaseSyncAngle" : {
"nodetype" : "namednumber",
"number" : "38"
},
"inletPhaseSync" : {
"nodetype" : "namednumber",
"number" : "39"
},
"operatingState" : {
"nodetype" : "namednumber",
"number" : "40"
},
"activeInlet" : {
"nodetype" : "namednumber",
"number" : "41"
},
"illuminance" : {
"nodetype" : "namednumber",
"number" : "42"
},
"doorContact" : {
"nodetype" : "namednumber",
"number" : "43"
},
"tamperDetection" : {
"nodetype" : "namednumber",
"number" : "44"
},
"motionDetection" : {
"nodetype" : "namednumber",
"number" : "45"
},
"i1smpsStatus" : {
"nodetype" : "namednumber",
"number" : "46"
},
"i2smpsStatus" : {
"nodetype" : "namednumber",
"number" : "47"
},
"switchStatus" : {
"nodetype" : "namednumber",
"number" : "48"
},
"description" :
"""The types a sensor can be.""",
},
"SensorStateEnumeration" : {
"basetype" : "Enumeration",
"status" : "current",
"unavailable" : {
"nodetype" : "namednumber",
"number" : "-1"
},
"open" : {
"nodetype" : "namednumber",
"number" : "0"
},
"closed" : {
"nodetype" : "namednumber",
"number" : "1"
},
"belowLowerCritical" : {
"nodetype" : "namednumber",
"number" : "2"
},
"belowLowerWarning" : {
"nodetype" : "namednumber",
"number" : "3"
},
"normal" : {
"nodetype" : "namednumber",
"number" : "4"
},
"aboveUpperWarning" : {
"nodetype" : "namednumber",
"number" : "5"
},
"aboveUpperCritical" : {
"nodetype" : "namednumber",
"number" : "6"
},
"on" : {
"nodetype" : "namednumber",
"number" : "7"
},
"off" : {
"nodetype" : "namednumber",
"number" : "8"
},
"detected" : {
"nodetype" : "namednumber",
"number" : "9"
},
"notDetected" : {
"nodetype" : "namednumber",
"number" : "10"
},
"alarmed" : {
"nodetype" : "namednumber",
"number" : "11"
},
"ok" : {
"nodetype" : "namednumber",
"number" : "12"
},
"fail" : {
"nodetype" : "namednumber",
"number" : "14"
},
"yes" : {
"nodetype" : "namednumber",
"number" : "15"
},
"no" : {
"nodetype" : "namednumber",
"number" : "16"
},
"standby" : {
"nodetype" : "namednumber",
"number" : "17"
},
"one" : {
"nodetype" : "namednumber",
"number" : "18"
},
"two" : {
"nodetype" : "namednumber",
"number" : "19"
},
"inSync" : {
"nodetype" : "namednumber",
"number" : "20"
},
"outOfSync" : {
"nodetype" : "namednumber",
"number" : "21"
},
"i1OpenFault" : {
"nodetype" : "namednumber",
"number" : "22"
},
"i1ShortFault" : {
"nodetype" : "namednumber",
"number" : "23"
},
"i2OpenFault" : {
"nodetype" : "namednumber",
"number" : "24"
},
"i2ShortFault" : {
"nodetype" : "namednumber",
"number" : "25"
},
"fault" : {
"nodetype" : "namednumber",
"number" : "26"
},
"warning" : {
"nodetype" : "namednumber",
"number" : "27"
},
"critical" : {
"nodetype" : "namednumber",
"number" : "28"
},
"selfTest" : {
"nodetype" : "namednumber",
"number" : "29"
},
"nonRedundant" : {
"nodetype" : "namednumber",
"number" : "30"
},
"description" :
"""The states a sensor can be in.""",
},
"PlugTypeEnumeration" : {
"basetype" : "Enumeration",
"status" : "current",
"plugOTHER" : {
"nodetype" : "namednumber",
"number" : "-1"
},
"plugNONE" : {
"nodetype" : "namednumber",
"number" : "0"
},
"plug56P320" : {
"nodetype" : "namednumber",
"number" : "1"
},
"plug56P520" : {
"nodetype" : "namednumber",
"number" : "2"
},
"plug56P532" : {
"nodetype" : "namednumber",
"number" : "3"
},
"plugCS8365C" : {
"nodetype" : "namednumber",
"number" : "4"
},
"plugIEC320C14" : {
"nodetype" : "namednumber",
"number" : "5"
},
"plugIEC320C20" : {
"nodetype" : "namednumber",
"number" : "6"
},
"plugIEC603093WIRE250V16A" : {
"nodetype" : "namednumber",
"number" : "7"
},
"plugIEC603093WIRE250V20A" : {
"nodetype" : "namednumber",
"number" : "8"
},
"plugIEC603093WIRE250V30A" : {
"nodetype" : "namednumber",
"number" : "9"
},
"plugIEC603093WIRE250V32A" : {
"nodetype" : "namednumber",
"number" : "10"
},
"plugIEC603093WIRE250V60A" : {
"nodetype" : "namednumber",
"number" : "11"
},
"plugIEC603093WIRE250V63A" : {
"nodetype" : "namednumber",
"number" : "12"
},
"plugIEC603093WIRE250V100A" : {
"nodetype" : "namednumber",
"number" : "13"
},
"plugIEC603093WIRE250V125A" : {
"nodetype" : "namednumber",
"number" : "14"
},
"plugIEC603094WIRE250V20A" : {
"nodetype" : "namednumber",
"number" : "15"
},
"plugIEC603094WIRE250V30A" : {
"nodetype" : "namednumber",
"number" : "16"
},
"plugIEC603094WIRE250V60A" : {
"nodetype" : "namednumber",
"number" : "17"
},
"plugIEC603094WIRE250V100A" : {
"nodetype" : "namednumber",
"number" : "18"
},
"plugIEC603095WIRE208V20A" : {
"nodetype" : "namednumber",
"number" : "23"
},
"plugIEC603095WIRE208V30A" : {
"nodetype" : "namednumber",
"number" : "24"
},
"plugIEC603095WIRE208V60A" : {
"nodetype" : "namednumber",
"number" : "25"
},
"plugIEC603095WIRE208V100A" : {
"nodetype" : "namednumber",
"number" : "26"
},
"plugIEC603095WIRE415V16A" : {
"nodetype" : "namednumber",
"number" : "27"
},
"plugIEC603095WIRE415V32A" : {
"nodetype" : "namednumber",
"number" : "28"
},
"plugIEC603095WIRE415V63A" : {
"nodetype" : "namednumber",
"number" : "29"
},
"plugIEC603095WIRE415V125A" : {
"nodetype" : "namednumber",
"number" : "30"
},
"plugIEC603095WIRE480V20A" : {
"nodetype" : "namednumber",
"number" : "31"
},
"plugIEC603095WIRE480V30A" : {
"nodetype" : "namednumber",
"number" : "32"
},
"plugIEC603095WIRE480V60A" : {
"nodetype" : "namednumber",
"number" : "33"
},
"plugIEC603095WIRE480V100A" : {
"nodetype" : "namednumber",
"number" : "34"
},
"plugNEMA515P" : {
"nodetype" : "namednumber",
"number" : "35"
},
"plugNEMAL515P" : {
"nodetype" : "namednumber",
"number" : "36"
},
"plugNEMA520P" : {
"nodetype" : "namednumber",
"number" : "37"
},
"plugNEMAL520P" : {
"nodetype" : "namednumber",
"number" : "38"
},
"plugNEMAL530P" : {
"nodetype" : "namednumber",
"number" : "39"
},
"plugNEMAL615P" : {
"nodetype" : "namednumber",
"number" : "40"
},
"plugNEMAL620P" : {
"nodetype" : "namednumber",
"number" : "41"
},
"plugNEMAL630P" : {
"nodetype" : "namednumber",
"number" : "42"
},
"plugNEMAL1520P" : {
"nodetype" : "namednumber",
"number" : "43"
},
"plugNEMAL1530P" : {
"nodetype" : "namednumber",
"number" : "44"
},
"plugNEMAL2120P" : {
"nodetype" : "namednumber",
"number" : "45"
},
"plugNEMAL2130P" : {
"nodetype" : "namednumber",
"number" : "46"
},
"plugNEMAL2230P" : {
"nodetype" : "namednumber",
"number" : "47"
},
"plug56P320F" : {
"nodetype" : "namednumber",
"number" : "48"
},
"plug56PA320" : {
"nodetype" : "namednumber",
"number" : "49"
},
"description" :
"""The types of inlet plug.""",
},
"ReceptacleTypeEnumeration" : {
"basetype" : "Enumeration",
"status" : "current",
"receptacleOTHER" : {
"nodetype" : "namednumber",
"number" : "-1"
},
"receptacleNONE" : {
"nodetype" : "namednumber",
"number" : "0"
},
"receptacleBS1363" : {
"nodetype" : "namednumber",
"number" : "1"
},
"receptacle56P532" : {
"nodetype" : "namednumber",
"number" : "3"
},
"receptacleCS8364C" : {
"nodetype" : "namednumber",
"number" : "4"
},
"receptacleIEC320C13" : {
"nodetype" : "namednumber",
"number" : "5"
},
"receptacleIEC320C19" : {
"nodetype" : "namednumber",
"number" : "6"
},
"receptacleIEC603093WIRE250V16A" : {
"nodetype" : "namednumber",
"number" : "7"
},
"receptacleIEC603093WIRE250V20A" : {
"nodetype" : "namednumber",
"number" : "8"
},
"receptacleIEC603093WIRE250V30A" : {
"nodetype" : "namednumber",
"number" : "9"
},
"receptacleIEC603093WIRE250V32A" : {
"nodetype" : "namednumber",
"number" : "10"
},
"receptacleIEC603093WIRE250V60A" : {
"nodetype" : "namednumber",
"number" : "11"
},
"receptacleIEC603093WIRE250V63A" : {
"nodetype" : "namednumber",
"number" : "12"
},
"receptacleIEC603093WIRE250V100A" : {
"nodetype" : "namednumber",
"number" : "13"
},
"receptacleIEC603093WIRE250V125A" : {
"nodetype" : "namednumber",
"number" : "14"
},
"receptacleIEC603094WIRE250V20A" : {
"nodetype" : "namednumber",
"number" : "15"
},
"receptacleIEC603094WIRE250V30A" : {
"nodetype" : "namednumber",
"number" : "16"
},
"receptacleIEC603094WIRE250V60A" : {
"nodetype" : "namednumber",
"number" : "17"
},
"receptacleIEC603094WIRE250V100A" : {
"nodetype" : "namednumber",
"number" : "18"
},
"receptacleIEC603095WIRE208V20A" : {
"nodetype" : "namednumber",
"number" : "23"
},
"receptacleIEC603095WIRE208V30A" : {
"nodetype" : "namednumber",
"number" : "24"
},
"receptacleIEC603095WIRE208V60A" : {
"nodetype" : "namednumber",
"number" : "25"
},
"receptacleIEC603095WIRE208V100A" : {
"nodetype" : "namednumber",
"number" : "26"
},
"receptacleIEC603095WIRE415V16A" : {
"nodetype" : "namednumber",
"number" : "27"
},
"receptacleIEC603095WIRE415V32A" : {
"nodetype" : "namednumber",
"number" : "28"
},
"receptacleIEC603095WIRE415V63A" : {
"nodetype" : "namednumber",
"number" : "29"
},
"receptacleIEC603095WIRE415V125A" : {
"nodetype" : "namednumber",
"number" : "30"
},
"receptacleIEC603095WIRE480V20A" : {
"nodetype" : "namednumber",
"number" : "31"
},
"receptacleIEC603095WIRE480V30A" : {
"nodetype" : "namednumber",
"number" : "32"
},
"receptacleIEC603095WIRE480V60A" : {
"nodetype" : "namednumber",
"number" : "33"
},
"receptacleIEC603095WIRE480V100A" : {
"nodetype" : "namednumber",
"number" : "34"
},
"receptacleNEMA515R" : {
"nodetype" : "namednumber",
"number" : "35"
},
"receptacleNEMAL515R" : {
"nodetype" : "namednumber",
"number" : "36"
},
"receptacleNEMA520R" : {
"nodetype" : "namednumber",
"number" : "37"
},
"receptacleNEMAL520R" : {
"nodetype" : "namednumber",
"number" : "38"
},
"receptacleNEMAL530R" : {
"nodetype" : "namednumber",
"number" : "39"
},
"receptacleNEMAL615R" : {
"nodetype" : "namednumber",
"number" : "40"
},
"receptacleNEMAL620R" : {
"nodetype" : "namednumber",
"number" : "41"
},
"receptacleNEMAL630R" : {
"nodetype" : "namednumber",
"number" : "42"
},
"receptacleNEMAL1520R" : {
"nodetype" : "namednumber",
"number" : "43"
},
"receptacleNEMAL1530R" : {
"nodetype" : "namednumber",
"number" : "44"
},
"receptacleNEMAL2120RP" : {
"nodetype" : "namednumber",
"number" : "45"
},
"receptacleNEMAL2130R" : {
"nodetype" : "namednumber",
"number" : "46"
},
"receptacleSCHUKOTYPEE" : {
"nodetype" : "namednumber",
"number" : "47"
},
"receptacleSCHUKOTYPEF" : {
"nodetype" : "namednumber",
"number" : "48"
},
"description" :
"""The types of outlet receptacle.""",
},
"OverCurrentProtectorTypeEnumeration" : {
"basetype" : "Enumeration",
"status" : "current",
"ocpBREAKER1POLE" : {
"nodetype" : "namednumber",
"number" : "1"
},
"ocpBREAKER2POLE" : {
"nodetype" : "namednumber",
"number" : "2"
},
"ocpBREAKER3POLE" : {
"nodetype" : "namednumber",
"number" : "3"
},
"ocpFUSE" : {
"nodetype" : "namednumber",
"number" : "4"
},
"ocpFUSEPAIR" : {
"nodetype" : "namednumber",
"number" : "5"
},
"ocpRCBO2POLE" : {
"nodetype" : "namednumber",
"number" : "6"
},
"ocpRCBO3POLE" : {
"nodetype" : "namednumber",
"number" : "7"
},
"ocpRCBO4POLE" : {
"nodetype" : "namednumber",
"number" : "8"
},
"description" :
"""The types of overcurrent protectors.""",
},
"BoardTypeEnumeration" : {
"basetype" : "Enumeration",
"status" : "current",
"mainController" : {
"nodetype" : "namednumber",
"number" : "1"
},
"inletController" : {
"nodetype" : "namednumber",
"number" : "2"
},
"outletController" : {
"nodetype" : "namednumber",
"number" : "3"
},
"meteringController" : {
"nodetype" : "namednumber",
"number" : "4"
},
"description" :
"""The types of boards.""",
},
"OutletSwitchingOperationsEnumeration" : {
"basetype" : "Enumeration",
"status" : "current",
"off" : {
"nodetype" : "namednumber",
"number" : "0"
},
"on" : {
"nodetype" : "namednumber",
"number" : "1"
},
"cycle" : {
"nodetype" : "namednumber",
"number" : "2"
},
"description" :
"""The switching operations on an outlet.""",
},
"SensorUnitsEnumeration" : {
"basetype" : "Enumeration",
"status" : "current",
"none" : {
"nodetype" : "namednumber",
"number" : "-1"
},
"other" : {
"nodetype" : "namednumber",
"number" : "0"
},
"volt" : {
"nodetype" : "namednumber",
"number" : "1"
},
"amp" : {
"nodetype" : "namednumber",
"number" : "2"
},
"watt" : {
"nodetype" : "namednumber",
"number" : "3"
},
"voltamp" : {
"nodetype" : "namednumber",
"number" : "4"
},
"wattHour" : {
"nodetype" : "namednumber",
"number" : "5"
},
"voltampHour" : {
"nodetype" : "namednumber",
"number" : "6"
},
"degreeC" : {
"nodetype" : "namednumber",
"number" : "7"
},
"hertz" : {
"nodetype" : "namednumber",
"number" : "8"
},
"percent" : {
"nodetype" : "namednumber",
"number" : "9"
},
"meterpersec" : {
"nodetype" : "namednumber",
"number" : "10"
},
"pascal" : {
"nodetype" : "namednumber",
"number" : "11"
},
"psi" : {
"nodetype" : "namednumber",
"number" : "12"
},
"g" : {
"nodetype" : "namednumber",
"number" : "13"
},
"degreeF" : {
"nodetype" : "namednumber",
"number" : "14"
},
"feet" : {
"nodetype" : "namednumber",
"number" : "15"
},
"inches" : {
"nodetype" : "namednumber",
"number" : "16"
},
"cm" : {
"nodetype" : "namednumber",
"number" : "17"
},
"meters" : {
"nodetype" : "namednumber",
"number" : "18"
},
"rpm" : {
"nodetype" : "namednumber",
"number" : "19"
},
"degrees" : {
"nodetype" : "namednumber",
"number" : "20"
},
"lux" : {
"nodetype" : "namednumber",
"number" : "21"
},
"grampercubicmeter" : {
"nodetype" : "namednumber",
"number" : "22"
},
"var" : {
"nodetype" : "namednumber",
"number" : "23"
},
"description" :
"""The sensor units.""",
},
"DaisychainMemberTypeEnumeration" : {
"basetype" : "Enumeration",
"status" : "current",
"standalone" : {
"nodetype" : "namednumber",
"number" : "0"
},
"master" : {
"nodetype" : "namednumber",
"number" : "1"
},
"slave" : {
"nodetype" : "namednumber",
"number" : "2"
},
"description" :
"""The daisy chain member type.""",
},
"URL" : {
"basetype" : "OctetString",
"status" : "current",
"ranges" : [
{
"min" : "0",
"max" : "255"
},
],
"range" : {
"min" : "0",
"max" : "255"
},
"format" : "255a",
"description" :
"""A Uniform Resource Locator (URL), as defined in RFC1738.""",
},
"GlobalOutletStateOnStartupEnumeration" : {
"basetype" : "Enumeration",
"status" : "current",
"off" : {
"nodetype" : "namednumber",
"number" : "0"
},
"on" : {
"nodetype" : "namednumber",
"number" : "1"
},
"lastKnownState" : {
"nodetype" : "namednumber",
"number" : "2"
},
"description" :
"""The global outlet state on device start up; can be overridden per outlet.""",
},
"OutletStateOnStartupEnumeration" : {
"basetype" : "Enumeration",
"status" : "current",
"off" : {
"nodetype" : "namednumber",
"number" : "0"
},
"on" : {
"nodetype" : "namednumber",
"number" : "1"
},
"lastKnownState" : {
"nodetype" : "namednumber",
"number" : "2"
},
"globalOutletStateOnStartup" : {
"nodetype" : "namednumber",
"number" : "3"
},
"description" :
"""The outlet state on device start up; this overrides the global value.""",
},
"ExternalSensorsZCoordinateUnitsEnumeration" : {
"basetype" : "Enumeration",
"status" : "current",
"rackUnits" : {
"nodetype" : "namednumber",
"number" : "0"
},
"text" : {
"nodetype" : "namednumber",
"number" : "1"
},
"description" :
"""The units of the external Sensor Z Coordinate.
rackUnits implies that the Z Coordinate for all external sensors
is in rack Units (U)
text implies that the Z Coordinate for all external sensors
is a text string (label) """,
},
"HundredthsOfAPercentage" : {
"basetype" : "Unsigned32",
"status" : "current",
"ranges" : [
{
"min" : "0",
"max" : "10000"
},
],
"range" : {
"min" : "0",
"max" : "10000"
},
"format" : "d",
"description" :
"""Data type for reporting values in hundredths of percentage, i.e. 0.01 %.""",
},
"DeviceIdentificationParameterEnumeration" : {
"basetype" : "Enumeration",
"status" : "current",
"pduName" : {
"nodetype" : "namednumber",
"number" : "0"
},
"sysContact" : {
"nodetype" : "namednumber",
"number" : "1"
},
"sysName" : {
"nodetype" : "namednumber",
"number" : "2"
},
"sysLocation" : {
"nodetype" : "namednumber",
"number" : "3"
},
"description" :
"""The configurable parameters.""",
},
"TransferSwitchTransferReasonEnumeration" : {
"basetype" : "Enumeration",
"status" : "current",
"unknown" : {
"nodetype" : "namednumber",
"number" : "0"
},
"startup" : {
"nodetype" : "namednumber",
"number" : "1"
},
"manualTransfer" : {
"nodetype" : "namednumber",
"number" : "2"
},
"automaticReTransfer" : {
"nodetype" : "namednumber",
"number" : "3"
},
"powerFailure" : {
"nodetype" : "namednumber",
"number" : "4"
},
"powerQuality" : {
"nodetype" : "namednumber",
"number" : "5"
},
"overloadAlarm" : {
"nodetype" : "namednumber",
"number" : "6"
},
"overheatAlarm" : {
"nodetype" : "namednumber",
"number" : "7"
},
"internalFailure" : {
"nodetype" : "namednumber",
"number" : "8"
},
"description" :
"""Transfer Switch Transfer Reason""",
},
"ProductTypeEnumeration" : {
"basetype" : "Enumeration",
"status" : "current",
"rackPdu" : {
"nodetype" : "namednumber",
"number" : "0"
},
"bcm" : {
"nodetype" : "namednumber",
"number" : "1"
},
"transferSwitch" : {
"nodetype" : "namednumber",
"number" : "2"
},
"powerMeter" : {
"nodetype" : "namednumber",
"number" : "3"
},
"description" :
"""The product types.""",
},
"RelayPowerLossBehaviorEnumeration" : {
"basetype" : "Enumeration",
"status" : "current",
"nonLatching" : {
"nodetype" : "namednumber",
"number" : "0"
},
"latching" : {
"nodetype" : "namednumber",
"number" : "1"
},
"description" :
"""The type of relay behavior on power loss.""",
},
"DeviceCascadeTypeEnumeration" : {
"basetype" : "Enumeration",
"status" : "current",
"bridging" : {
"nodetype" : "namednumber",
"number" : "0"
},
"portForwarding" : {
"nodetype" : "namednumber",
"number" : "1"
},
"description" :
"""The type of configured cascading on this device.""",
},
"PeripheralDeviceFirmwareUpdateStateEnumeration" : {
"basetype" : "Enumeration",
"status" : "current",
"started" : {
"nodetype" : "namednumber",
"number" : "1"
},
"successful" : {
"nodetype" : "namednumber",
"number" : "2"
},
"failed" : {
"nodetype" : "namednumber",
"number" : "3"
},
"description" :
"""The state of an peripheral device firmware update.""",
},
"PanelLayoutEnumeration" : {
"basetype" : "Enumeration",
"status" : "current",
"invalid" : {
"nodetype" : "namednumber",
"number" : "-1"
},
"oneColumn" : {
"nodetype" : "namednumber",
"number" : "1"
},
"twoColumns" : {
"nodetype" : "namednumber",
"number" : "2"
},
"description" :
"""The panel circuit position layout.""",
},
"PanelNumberingEnumeration" : {
"basetype" : "Enumeration",
"status" : "current",
"invalid" : {
"nodetype" : "namednumber",
"number" : "-1"
},
"oddEven" : {
"nodetype" : "namednumber",
"number" : "1"
},
"sequential" : {
"nodetype" : "namednumber",
"number" : "2"
},
"description" :
"""The panel circuit position numbering scheme.""",
},
"CircuitTypeEnumeration" : {
"basetype" : "Enumeration",
"status" : "current",
"onePhaseLL" : {
"nodetype" : "namednumber",
"number" : "1"
},
"onePhaseLN" : {
"nodetype" : "namednumber",
"number" : "2"
},
"onePhaseLLN" : {
"nodetype" : "namednumber",
"number" : "3"
},
"threePhase" : {
"nodetype" : "namednumber",
"number" : "4"
},
"description" :
"""The panel circuit types.""",
},
"PhaseEnumeration" : {
"basetype" : "Enumeration",
"status" : "current",
"phaseA" : {
"nodetype" : "namednumber",
"number" : "1"
},
"phaseB" : {
"nodetype" : "namednumber",
"number" : "2"
},
"phaseC" : {
"nodetype" : "namednumber",
"number" : "3"
},
"neutral" : {
"nodetype" : "namednumber",
"number" : "4"
},
"earth" : {
"nodetype" : "namednumber",
"number" : "5"
},
"description" :
"""The power phase.""",
},
"LineEnumeration" : {
"basetype" : "Enumeration",
"status" : "current",
"lineL1" : {
"nodetype" : "namednumber",
"number" : "1"
},
"lineL2" : {
"nodetype" : "namednumber",
"number" : "2"
},
"lineL3" : {
"nodetype" : "namednumber",
"number" : "3"
},
"lineNeutral" : {
"nodetype" : "namednumber",
"number" : "4"
},
"description" :
"""The Lines: L1, L2, L3, N.""",
},
"PowerMeterTypeEnumeration" : {
"basetype" : "Enumeration",
"status" : "current",
"singlePhase" : {
"nodetype" : "namednumber",
"number" : "1"
},
"splitPhase" : {
"nodetype" : "namednumber",
"number" : "2"
},
"threePhase" : {
"nodetype" : "namednumber",
"number" : "3"
},
"description" :
"""The power meter types""",
},
"NetworkInterfaceTypeEnumeration" : {
"basetype" : "Enumeration",
"status" : "current",
"wired" : {
"nodetype" : "namednumber",
"number" : "0"
},
"wireless" : {
"nodetype" : "namednumber",
"number" : "1"
},
"description" :
"""The type of network interface.""",
},
"AddressSourceEnumeration" : {
"basetype" : "Enumeration",
"status" : "current",
"static" : {
"nodetype" : "namednumber",
"number" : "1"
},
"dhcp" : {
"nodetype" : "namednumber",
"number" : "2"
},
"dhcpv6" : {
"nodetype" : "namednumber",
"number" : "3"
},
"description" :
"""How was the address obtained?""",
},
}, # typedefs
"nodes" : {
"raritan" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742",
"status" : "current",
}, # node
"pdu2" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6",
}, # node
"traps" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0",
}, # node
"trapInformation" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.0",
}, # node
"trapInformationTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.0.1",
"status" : "current",
"description" :
"""A list of Trap Information entries. The number of
entries is given by the value of pduCount.""",
}, # table
"trapInformationEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.0.1.1",
"status" : "current",
"linkage" : [
"pduId",
],
"description" :
"""An entry containing objects used in traps.""",
}, # row
"userName" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.0.1.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The user currently logged in.""",
}, # column
"targetUser" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.0.1.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The user added, deleted, or modified.""",
}, # column
"imageVersion" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.0.1.1.5",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The version of the upgrade image.""",
}, # column
"roleName" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.0.1.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The role added, deleted, or modified.""",
}, # column
"smtpMessageRecipients" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.0.1.1.7",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""Comma separated list of SMTP Message recipients""",
}, # column
"smtpServer" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.0.1.1.8",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The IP address/host name of the SMTP server""",
}, # column
"oldSensorState" : {
"nodetype" : "scalar",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.0.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorStateEnumeration"},
},
"access" : "notifyonly",
"description" :
"""The old sensor state used in Sensor State Transition traps.""",
}, # scalar
"pduNumber" : {
"nodetype" : "scalar",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.0.3",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "256"
},
],
"range" : {
"min" : "1",
"max" : "256"
},
},
},
"access" : "notifyonly",
"description" :
"""A unique value for each PDU. Its value
ranges between 1 and the value of pduCount.""",
}, # scalar
"inletPoleNumber" : {
"nodetype" : "scalar",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.0.5",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "256"
},
],
"range" : {
"min" : "1",
"max" : "256"
},
},
},
"access" : "notifyonly",
"description" :
"""A unique value for each inlet Pole. Its value
ranges between 1 and the value of inletPoleCount.""",
}, # scalar
"outletPoleNumber" : {
"nodetype" : "scalar",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.0.7",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "256"
},
],
"range" : {
"min" : "1",
"max" : "256"
},
},
},
"access" : "notifyonly",
"description" :
"""A unique value for each outlet Pole. Its value
ranges between 1 and the value of outletPoleCount.""",
}, # scalar
"externalSensorNumber" : {
"nodetype" : "scalar",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.0.8",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "256"
},
],
"range" : {
"min" : "1",
"max" : "256"
},
},
},
"access" : "notifyonly",
"description" :
"""A unique value for each external sensor. Its value
ranges between 1 and the value of externalSensorCount.""",
}, # scalar
"typeOfSensor" : {
"nodetype" : "scalar",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.0.10",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorTypeEnumeration"},
},
"access" : "notifyonly",
"description" :
"""The type of sensor.""",
}, # scalar
"errorDescription" : {
"nodetype" : "scalar",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.0.11",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "notifyonly",
"description" :
"""Description of the Error""",
}, # scalar
"deviceChangedParameter" : {
"nodetype" : "scalar",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.0.12",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "DeviceIdentificationParameterEnumeration"},
},
"access" : "notifyonly",
"description" :
"""Description of the parameter(s) that changed.""",
}, # scalar
"changedParameterNewValue" : {
"nodetype" : "scalar",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.0.13",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "notifyonly",
"description" :
"""The new value of the changed parameter """,
}, # scalar
"lhxSupportEnabled" : {
"nodetype" : "scalar",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.0.14",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "notifyonly",
"description" :
"""The new enabled state for Schroff LHX Support.""",
}, # scalar
"webcamModel" : {
"nodetype" : "scalar",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.0.15",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "notifyonly",
"description" :
"""The model of the Webcam """,
}, # scalar
"webcamConnectionPort" : {
"nodetype" : "scalar",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.0.16",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "notifyonly",
"description" :
"""The port to which the Webcam is connected """,
}, # scalar
"agentInetPortNumber" : {
"nodetype" : "scalar",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.0.18",
"status" : "current",
"syntax" : {
"type" : { "module" :"INET-ADDRESS-MIB", "name" : "InetPortNumber"},
},
"access" : "notifyonly",
"description" :
"""The UDP port number used for accessing
the SNMP agent on the device.
Examples:
If deviceCascadeType is portForwarding, then
master: 50500
slave 1:50501
slave 2: 50502
......
If cascading mode is not portForwarding and default (Standard) ports are being used, then
port: 161
""",
}, # scalar
"peripheralDeviceRomcode" : {
"nodetype" : "scalar",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.0.19",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "notifyonly",
"description" :
"""The Romcode of an peripheral device""",
}, # scalar
"peripheralDeviceFirmwareUpdateState" : {
"nodetype" : "scalar",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.0.20",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "PeripheralDeviceFirmwareUpdateStateEnumeration"},
},
"access" : "notifyonly",
"description" :
"""The firmware update state of an peripheral device""",
}, # scalar
"circuitNumber" : {
"nodetype" : "scalar",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.0.21",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "33000"
},
],
"range" : {
"min" : "1",
"max" : "33000"
},
},
},
"access" : "notifyonly",
"description" :
"""A unique value for each circuit.
circuitId is defined as follows.
circuitID = 1000*(panelId) + circuitPosition
Examples:
1045 is the the circuit on panel 1 with lowest circuit position equal to 45
4067 is the the circuit on panel 4 with lowest circuit position equal to 67. """,
}, # scalar
"circuitPoleNumber" : {
"nodetype" : "scalar",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.0.22",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "256"
},
],
"range" : {
"min" : "1",
"max" : "256"
},
},
},
"access" : "notifyonly",
"description" :
"""A unique value for each circuit Pole. """,
}, # scalar
"phoneNumber" : {
"nodetype" : "scalar",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.0.23",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "notifyonly",
"description" :
"""The phone number of e.g. an SMS receiver.""",
}, # scalar
"board" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.1",
}, # node
"environmental" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.2",
}, # node
"configuration" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3",
}, # node
"pduCount" : {
"nodetype" : "scalar",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.1",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""For a PX2/3 and transfer switch, pduCount = 1
For a BCM2, pduCount = number of power meters + 1 (for the main controller)""",
}, # scalar
"unit" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2",
}, # node
"nameplateTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.1",
"status" : "current",
"description" :
"""A list of PDU nameplate entries. The number of
entries is given by the value of pduCount.""",
}, # table
"nameplateEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.1.1",
"status" : "current",
"linkage" : [
"pduId",
],
"description" :
"""An entry providing PDU nameplate information.""",
}, # row
"pduId" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.1.1.1",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "0",
"max" : "256"
},
],
"range" : {
"min" : "0",
"max" : "256"
},
},
},
"access" : "noaccess",
"description" :
"""A unique value for each PDU/Power meter.
PX2/3 and transfer switch: pduiId = 1
BCM2:
main controller: pduId = 0
power meter: pduId = rotary switch setting for the power meter
It is the same as the MeterID in the GUI
Example:
a power meter, rotary switch setting = 5, pduId = 5
a panel (power meter + branch metering), rotary switch setting = 23, pduId = 23 """,
}, # column
"pduManufacturer" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.1.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The PDU manaufacturer.""",
}, # column
"pduModel" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.1.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The PDU model.""",
}, # column
"pduSerialNumber" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.1.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The PDU serial Number.""",
}, # column
"pduRatedVoltage" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.1.1.5",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The PDU voltage rating.""",
}, # column
"pduRatedCurrent" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.1.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The PDU current rating.""",
}, # column
"pduRatedFrequency" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.1.1.7",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The PDU frequency rating.""",
}, # column
"pduRatedVA" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.1.1.8",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The PDU VA (VoltAmps) rating.""",
}, # column
"pduImage" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.1.1.9",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "URL"},
},
"access" : "readonly",
"description" :
"""The URL of the wiring diagram for this PDU.""",
}, # column
"unitConfigurationTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2",
"status" : "current",
"description" :
"""A list of PDU configuration entries. The number of
entries is given by the value of pduCount.""",
}, # table
"unitConfigurationEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1",
"status" : "current",
"linkage" : [
"pduId",
],
"description" :
"""An entry containing configuration objects for a particular PDU.""",
}, # row
"inletCount" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.2",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "256"
},
],
"range" : {
"min" : "1",
"max" : "256"
},
},
},
"access" : "readonly",
"description" :
"""The number of inlets.""",
}, # column
"overCurrentProtectorCount" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.3",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "0",
"max" : "255"
},
],
"range" : {
"min" : "0",
"max" : "255"
},
},
},
"access" : "readonly",
"description" :
"""The number of overcurrent protectors.""",
}, # column
"outletCount" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.4",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "256"
},
],
"range" : {
"min" : "1",
"max" : "256"
},
},
},
"access" : "readonly",
"description" :
"""The number of outlets.""",
}, # column
"inletControllerCount" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.5",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "0",
"max" : "255"
},
],
"range" : {
"min" : "0",
"max" : "255"
},
},
},
"access" : "readonly",
"description" :
"""The number of inlet controllers.""",
}, # column
"outletControllerCount" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.6",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "0",
"max" : "255"
},
],
"range" : {
"min" : "0",
"max" : "255"
},
},
},
"access" : "readonly",
"description" :
"""The number of outlet controllers.""",
}, # column
"externalSensorCount" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.7",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "0",
"max" : "255"
},
],
"range" : {
"min" : "0",
"max" : "255"
},
},
},
"access" : "readonly",
"description" :
"""The number of external sensors.""",
}, # column
"pxIPAddress" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.8",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"SNMPv2-SMI", "name" : "IpAddress"},
},
"access" : "readonly",
"description" :
"""The current IP address. A value of 0.0.0.0 indicates an error
or an unset option.""",
}, # column
"netmask" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.9",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"SNMPv2-SMI", "name" : "IpAddress"},
},
"access" : "readonly",
"description" :
"""The current netmask. A value of 0.0.0.0 indicates an error
or an unset option.""",
}, # column
"gateway" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.10",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"SNMPv2-SMI", "name" : "IpAddress"},
},
"access" : "readonly",
"description" :
"""The current gateway. A value of 0.0.0.0 indicates an error
or an unset option.""",
}, # column
"pxMACAddress" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.11",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "MacAddress"},
},
"access" : "readonly",
"description" :
"""The current MAC address.""",
}, # column
"utcOffset" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.12",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The current UTC offset.""",
}, # column
"pduName" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.13",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readwrite",
"description" :
"""The user-defined name for the PDU.""",
}, # column
"networkInterfaceType" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.14",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "NetworkInterfaceTypeEnumeration"},
},
"access" : "readonly",
"description" :
"""The network interface type: wired or wireless.""",
}, # column
"externalSensorsZCoordinateUnits" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.34",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "ExternalSensorsZCoordinateUnitsEnumeration"},
},
"access" : "readwrite",
"description" :
"""External Sensor Z Coordinate units: Freeform Text or Rack Units (U)
Default is U.""",
}, # column
"unitDeviceCapabilities" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.35",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Bits",
"i1smpsStatus" : {
"nodetype" : "namednumber",
"number" : "45"
},
"i2smpsStatus" : {
"nodetype" : "namednumber",
"number" : "46"
},
},
},
"access" : "readonly",
"description" :
"""A bit string which indicates which unit sensors are available.""",
}, # column
"outletSequencingDelay" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.36",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""It is deprecated. This is an alias for inrushGuardDelay""",
}, # column
"globalOutletPowerCyclingPowerOffPeriod" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.37",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The power-off period when an outlet is cycled;
applies to all outlets unless overridden at the outlet level;
specified in seconds;
0 <= value <= 3600 seconds.""",
}, # column
"globalOutletStateOnStartup" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.38",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "GlobalOutletStateOnStartupEnumeration"},
},
"access" : "readwrite",
"description" :
"""The outlet state on device startup; applies to all outlets
unless overridden at the outlet level.
Note that this value is ignored if relayBehaviorOnPowerLoss is set to latching.""",
}, # column
"outletPowerupSequence" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.39",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readwrite",
"description" :
"""The sequence in which will the outlets will be switched on under the following conditions.
1) Switch all outlets on operation is executed
2) Power to the PDU is cycled
String must consist of a comma separated sequence of the outlet numbers and
all outlet numbers must be included. The numbers entered must be a permutation of the numbers
1,2,3,-outletnumber.
Example for a 12 outlet PDU: 1,12,3,5,6,7,10,2,4,11,9,8.
The per outlet sequence delays are defined as outletSequenceDelay in the outletConfigurationTable""",
}, # column
"pduPowerCyclingPowerOffPeriod" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.40",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""When power to the PX is cycled (either manually or because of a
temporary power loss), this number determines how many seconds the
PX will wait before it provides power to the outlets.
specified in seconds:
0 <= value <= 3600 seconds.
Note that this value is ignored if relayBehaviorOnPowerLoss is set to latching.""",
}, # column
"pduDaisychainMemberType" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.41",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "DaisychainMemberTypeEnumeration"},
},
"access" : "readonly",
"description" :
"""The daisy chain member type.""",
}, # column
"managedExternalSensorCount" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.42",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "0",
"max" : "255"
},
],
"range" : {
"min" : "0",
"max" : "255"
},
},
},
"access" : "readonly",
"description" :
"""The number of managed external sensors """,
}, # column
"pxInetAddressType" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.50",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"INET-ADDRESS-MIB", "name" : "InetAddressType"},
},
"access" : "readonly",
"description" :
"""The type of address format
This object is deprecated in favor of ipAddressTable from the IP-MIB (rfc4293).""",
}, # column
"pxInetIPAddress" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.51",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"INET-ADDRESS-MIB", "name" : "InetAddress"},
},
"access" : "readonly",
"description" :
"""The current IP address. A value of 0.0.0.0 indicates an error
or an unset option.
This object is deprecated in favor of ipAddressTable from the IP-MIB (rfc4293).
For IPv6, its value is 0.0.0.0 """,
}, # column
"pxInetNetmask" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.52",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"INET-ADDRESS-MIB", "name" : "InetAddress"},
},
"access" : "readonly",
"description" :
"""The current netmask. A value of 0.0.0.0 indicates an error
or an unset option.
This object is deprecated in favor of ipAddressTable from the IP-MIB (rfc4293).
For IPv6, its value is 0.0.0.0 """,
}, # column
"pxInetGateway" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.53",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"INET-ADDRESS-MIB", "name" : "InetAddress"},
},
"access" : "readonly",
"description" :
"""The current gateway. A value of 0.0.0.0 indicates an error
or an unset option.
This object is deprecated in favor of ipAddressTable from the IP-MIB (rfc4293).
For IPv6, its value is 0.0.0.0 """,
}, # column
"loadShedding" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.55",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readwrite",
"description" :
"""Enter/Exit Load Shedding Mode""",
}, # column
"serverCount" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.56",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "0",
"max" : "255"
},
],
"range" : {
"min" : "0",
"max" : "255"
},
},
},
"access" : "readonly",
"description" :
"""The number of servers""",
}, # column
"inrushGuardDelay" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.57",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The time interval between switching on two outlets;
specified in milliseconds;
100 <= value <= 100000 milliseconds.""",
}, # column
"cascadedDeviceConnected" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.58",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readonly",
"description" :
"""Indicates whether another PX2 is connected using an USB cable to
the USB-A port of this PX2 in a cascaded configuration.
true: Connected
false: Not Connected""",
}, # column
"synchronizeWithNTPServer" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.59",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readwrite",
"description" :
"""Will time be obtained using NTP?
true: time will be obtained using NTP servers
false: time will not be obtained using NTP servers
Deafault is false.""",
}, # column
"useDHCPProvidedNTPServer" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.60",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readwrite",
"description" :
"""Will the NTP server addresses be provided by DHCP/BOOTP?
This is used only if synchronizeWithNTPServer is enabled
Default is enabled, i.e. DHCP provided NTP servers will be used """,
}, # column
"firstNTPServerAddressType" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.61",
"status" : "current",
"syntax" : {
"type" : { "module" :"INET-ADDRESS-MIB", "name" : "InetAddressType"},
},
"access" : "readwrite",
"default" : "ipv4",
"description" :
"""Represents the type of the corresponding instance
of firstNTPServerAddress object.""",
}, # column
"firstNTPServerAddress" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.62",
"status" : "current",
"syntax" : {
"type" : { "module" :"INET-ADDRESS-MIB", "name" : "InetAddress"},
},
"access" : "readwrite",
"description" :
"""The address of the primary ntp server.""",
}, # column
"secondNTPServerAddressType" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.63",
"status" : "current",
"syntax" : {
"type" : { "module" :"INET-ADDRESS-MIB", "name" : "InetAddressType"},
},
"access" : "readwrite",
"description" :
"""Represents the type of the corresponding instance
of secondNTPServerAddress object.
Default is ipv4""",
}, # column
"secondNTPServerAddress" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.64",
"status" : "current",
"syntax" : {
"type" : { "module" :"INET-ADDRESS-MIB", "name" : "InetAddress"},
},
"access" : "readwrite",
"description" :
"""The address of the second ntp server.""",
}, # column
"wireCount" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.65",
"status" : "deprecated",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "0",
"max" : "255"
},
],
"range" : {
"min" : "0",
"max" : "255"
},
},
},
"access" : "readonly",
"description" :
"""The number of wires.""",
}, # column
"transferSwitchCount" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.66",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "0",
"max" : "255"
},
],
"range" : {
"min" : "0",
"max" : "255"
},
},
},
"access" : "readonly",
"description" :
"""The number of transfer switches.""",
}, # column
"productType" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.67",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "ProductTypeEnumeration"},
},
"access" : "readonly",
"description" :
"""The product type.
Is this product a PDU, STS, BCM,...?""",
}, # column
"meteringControllerCount" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.68",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "0",
"max" : "255"
},
],
"range" : {
"min" : "0",
"max" : "255"
},
},
},
"access" : "readonly",
"description" :
"""The number of metering controllers.""",
}, # column
"relayBehaviorOnPowerLoss" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.69",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "RelayPowerLossBehaviorEnumeration"},
},
"access" : "readwrite",
"description" :
"""The relay behavior on power loss.""",
}, # column
"deviceCascadeType" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.70",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "DeviceCascadeTypeEnumeration"},
},
"access" : "readwrite",
"description" :
"""The configured type of cascading:
default is bridging.
This can be set only if the unit is the master.""",
}, # column
"deviceCascadePosition" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.71",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "0",
"max" : "99"
},
],
"range" : {
"min" : "0",
"max" : "99"
},
},
},
"access" : "readonly",
"description" :
"""The position of the device in the cascaded chain.
0: master
>= 1: slaves """,
}, # column
"peripheralDevicesAutoManagement" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.72",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readwrite",
"description" :
"""Auto-management enabled state for peripheral devices.""",
}, # column
"frontPanelOutletSwitching" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.73",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readwrite",
"description" :
"""Enables/disables switching of outlets using the PDU front panel.""",
}, # column
"frontPanelRCMSelfTest" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.74",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readwrite",
"description" :
"""Enables/disables front panel RCM self-test.""",
}, # column
"frontPanelActuatorControl" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.75",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readwrite",
"description" :
"""Enables/disables front panel peripheral actuator control.""",
}, # column
"circuitCount" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.76",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The number of user configured circuits in the panel.""",
}, # column
"activeDNSServerCount" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.2.1.77",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "0",
"max" : "16"
},
],
"range" : {
"min" : "0",
"max" : "16"
},
},
},
"access" : "readonly",
"description" :
"""The number of active DNS Servers """,
}, # column
"controllerConfigurationTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.3",
"status" : "current",
"description" :
"""A list of entries for the boards in each PDU. The number of
entries is given by the value of
inletControllerCount + outletControllerCount + 1 (for main controller board).""",
}, # table
"controllerConfigurationEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.3.1",
"status" : "current",
"linkage" : [
"pduId",
"boardType",
"boardIndex",
],
"description" :
"""An entry containing objects for a controller.""",
}, # row
"boardType" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.3.1.1",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "BoardTypeEnumeration"},
},
"access" : "noaccess",
"description" :
"""The type of board.""",
}, # column
"boardIndex" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.3.1.2",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "256"
},
],
"range" : {
"min" : "1",
"max" : "256"
},
},
},
"access" : "noaccess",
"description" :
"""A unique value for each controller. Its value
ranges between 1 and the value of
inletControllerCount + outletControllerCount + 1.""",
}, # column
"boardVersion" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.3.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The board hardware version.""",
}, # column
"boardFirmwareVersion" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.3.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The firmware version.""",
}, # column
"boardFirmwareTimeStamp" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.3.1.8",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The time when the board firmware was updated in UNIX(epoch)time.
It is measured in seconds relative to January 1, 1970 (midnight UTC/GMT),
i.e a value of 0 indicates January 1, 1970 (midnight UTC/GMT).""",
}, # column
"logConfigurationTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.4",
"status" : "current",
"description" :
"""A table of parameters for the data logging feature. The number of
entries is given by the value of pduCount.""",
}, # table
"logConfigurationEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.4.1",
"status" : "current",
"linkage" : [
"pduId",
],
"description" :
"""An entry containing data logging parameters
for a particular PDU.""",
}, # row
"dataLogging" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.4.1.1",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readwrite",
"description" :
"""Data Retrieval: enabled/disabled.""",
}, # column
"measurementPeriod" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.4.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""Data Collection periodicity. This is the periodicity of the data collected by the PX.
This value is fixed at 1 second.""",
}, # column
"measurementsPerLogEntry" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.4.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The number of measurements used for each entry in the log.""",
}, # column
"logSize" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.4.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The number of entries in the log.""",
}, # column
"dataLoggingEnableForAllSensors" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.4.1.5",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readwrite",
"description" :
"""Operation to control data logging for all sensors.
If this OID is set to true, then all xxxSensorLogAvailable are set to true
If this OID is set to false, then all xxxSensorLogAvailable are set to false""",
}, # column
"unitSensorConfigurationTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.5",
"status" : "current",
"description" :
"""A list of unit level sensors for a PDU.""",
}, # table
"unitSensorConfigurationEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.5.1",
"status" : "current",
"linkage" : [
"pduId",
"sensorType",
],
"description" :
"""An entry containing unit sensor parameters.""",
}, # row
"sensorType" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.5.1.1",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorTypeEnumeration"},
},
"access" : "noaccess",
"description" :
"""The type of sensor.""",
}, # column
"unitSensorLogAvailable" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.5.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readwrite",
"description" :
"""Is logging enabled for the sensor?""",
}, # column
"unitSensorUnits" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.5.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorUnitsEnumeration"},
},
"access" : "readonly",
"description" :
"""The units in which the sensor data is reported.""",
}, # column
"unitSensorDecimalDigits" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.5.1.7",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The number of digits displayed to the right of the decimal point.""",
}, # column
"unitSensorAccuracy" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.5.1.8",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "HundredthsOfAPercentage"},
},
"access" : "readonly",
"description" :
"""The accuracy: how close (in percent) the measurement is to the actual value.""",
}, # column
"unitSensorResolution" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.5.1.9",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The resolution: the minimum difference between any two measured values
The value of this OID variable should be scaled by
unitSensorDecimalDigits. For example, if the value is 1 and
unitSensorDecimalDigits is 2, then actual value is 0.01.""",
}, # column
"unitSensorTolerance" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.5.1.10",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The resolution: the difference between a sensor value and the actual value
The value of this OID variable should be scaled by
(unitSensorDecimalDigits + 1). For example, if the value is 50 and
unitSensorDecimalDigits is 2, then actual value is 0.05.""",
}, # column
"unitSensorMaximum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.5.1.11",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The largest possible reading as an unsigned integer.
The value of this OID variable should be scaled by
unitSensorDecimalDigits. For example, if the value is 1 and
unitSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled maximum exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"unitSensorMinimum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.5.1.12",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The smallest possible reading as an unsigned integer.
The value of this OID variable should be scaled by
unitSensorDecimalDigits. For example, if the value is 1 and
unitSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"unitSensorHysteresis" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.5.1.13",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The hysteresis used for deassertions
The value of this OID variable should be scaled by
unitSensorDecimalDigits. For example, if the value is 1 and
unitSensorDecimalDigits is 2, then actual value is 0.01.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"unitSensorStateChangeDelay" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.5.1.14",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The delay measured in samples before a state is asserted.
If the value is zero, then the state is asserted as soon as
it is detected; if it is non-zero, say n, then the assertion
condition must exist for n+1 consecutive samples before the corresponding
assertion event is reported.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"unitSensorLowerCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.5.1.21",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The lower critical threshold as an unsigned integer.
The value of this OID variable should be scaled by
unitSensorDecimalDigits. For example, if the value is 1 and
unitSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"unitSensorLowerWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.5.1.22",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The lower warning threshold as an unsigned integer.
The value of this OID variable should be scaled by
unitSensorDecimalDigits. For example, if the value is 1 and
unitSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"unitSensorUpperCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.5.1.23",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The upper critical threshold as an unsigned integer.
The value of this OID variable should be scaled by
unitSensorDecimalDigits. For example, if the value is 1 and
unitSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"unitSensorUpperWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.5.1.24",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The upper warning threshold as an unsigned integer.
The value of this OID variable should be scaled by
unitSensorDecimalDigits. For example, if the value is 1 and
unitSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"unitSensorEnabledThresholds" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.5.1.25",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Bits",
"lowerCritical" : {
"nodetype" : "namednumber",
"number" : "0"
},
"lowerWarning" : {
"nodetype" : "namednumber",
"number" : "1"
},
"upperWarning" : {
"nodetype" : "namednumber",
"number" : "2"
},
"upperCritical" : {
"nodetype" : "namednumber",
"number" : "3"
},
},
},
"access" : "readwrite",
"description" :
"""A bit string which indicates which thresholds are enabled.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"unitSensorSignedMaximum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.5.1.26",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The largest possible reading as a signed integer.
The value of this OID variable should be scaled by
unitSensorDecimalDigits. For example, if the value is 1 and
unitSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading.""",
}, # column
"unitSensorSignedMinimum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.5.1.27",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The smallest possible reading as a signed integer.
The value of this OID variable should be scaled by
unitSensorDecimalDigits. For example, if the value is 1 and
unitSensorDecimalDigits is 2, then actual value is 0.01.
This value determines whether to use the signed or unsigned threshold
and value columns: If the minimum is below zero the sensor can have
negative readings and the signed columns should be used.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"unitSensorSignedLowerCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.5.1.28",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The lower critical threshold as a signed integer.
The value of this OID variable should be scaled by
unitSensorDecimalDigits. For example, if the value is 1 and
unitSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading.""",
}, # column
"unitSensorSignedLowerWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.5.1.29",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The lower warning threshold as a signed integer.
The value of this OID variable should be scaled by
unitSensorDecimalDigits. For example, if the value is 1 and
unitSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading.""",
}, # column
"unitSensorSignedUpperCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.5.1.30",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The upper critical threshold as a signed integer.
The value of this OID variable should be scaled by
unitSensorDecimalDigits. For example, if the value is 1 and
unitSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading.""",
}, # column
"unitSensorSignedUpperWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.5.1.31",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The upper warning threshold as a signed integer.
The value of this OID variable should be scaled by
unitSensorDecimalDigits. For example, if the value is 1 and
unitSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading.""",
}, # column
"activeDNSServerTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.6",
"status" : "current",
"description" :
"""A list of active DNS server entries. The number of
rows in the table is given by the value of activeDNSServerCount (from unitConfigurationTable).
If activeDNSServerCount = 0, then the table is empty.""",
}, # table
"activeDNSServerEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.6.1",
"status" : "current",
"linkage" : [
"pduId",
"activeDNSServerIndex",
],
"description" :
"""An entry containing the active DNS servers for a particular PDU.""",
}, # row
"activeDNSServerIndex" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.6.1.2",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "16"
},
],
"range" : {
"min" : "1",
"max" : "16"
},
},
},
"access" : "noaccess",
"description" :
"""A unique value for a dns Server. Its value
ranges between 1 and activeDNSServerCount.""",
}, # column
"activeDNSServerAddressType" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.6.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"INET-ADDRESS-MIB", "name" : "InetAddressType"},
},
"access" : "readonly",
"description" :
"""Represents the type of the corresponding instance
of activeDNSServerAddress object.""",
}, # column
"activeDNSServerAddress" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.6.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"INET-ADDRESS-MIB", "name" : "InetAddress"},
},
"access" : "readonly",
"description" :
"""The address of the dns server.""",
}, # column
"activeDNSServerAddressSource" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.2.6.1.5",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "AddressSourceEnumeration"},
},
"access" : "readonly",
"description" :
"""How was the address obtained: static, dhcp, dhcpv6?""",
}, # column
"inlets" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3",
}, # node
"inletConfigurationTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.3",
"status" : "current",
"description" :
"""A list of inlet configuration entries. The number of
entries is given by the value of inletCount for the PDU.""",
}, # table
"inletConfigurationEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.3.1",
"status" : "current",
"linkage" : [
"pduId",
"inletId",
],
"description" :
"""An entry containing parametersfor a particular inlet.""",
}, # row
"inletId" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.3.1.1",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "256"
},
],
"range" : {
"min" : "1",
"max" : "256"
},
},
},
"access" : "noaccess",
"description" :
"""A unique value for each inlet. Its value
ranges between 1 and the value of inletCount.""",
}, # column
"inletLabel" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.3.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The label on the PDU identifying the inlet.""",
}, # column
"inletName" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.3.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readwrite",
"description" :
"""The user-defined name.""",
}, # column
"inletPlug" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.3.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "PlugTypeEnumeration"},
},
"access" : "readonly",
"description" :
"""The type of plug/receptacle wired to the inlet.""",
}, # column
"inletPoleCount" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.3.1.5",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "2",
"max" : "5"
},
],
"range" : {
"min" : "2",
"max" : "5"
},
},
},
"access" : "readonly",
"description" :
"""The number of poles.
PDU:
2 for 1-phase circuits
3 for 3-phase DELTA wired models;
4 for 3-phase WYE wired models; Pole 4 is neutral;
Note that sensors are not supported for neutral poles;
Power Meter:
5:
Pole 1 is Phase A
Pole 2 is Phase B
Pole 3 is Phase C
Pole 4 is Neutral
Pole 5 is Earth""",
}, # column
"inletRatedVoltage" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.3.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The inlet voltage rating.""",
}, # column
"inletRatedCurrent" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.3.1.7",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The inlet current rating.""",
}, # column
"inletRatedFrequency" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.3.1.8",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The inlet frequency rating.
Deprecated: use pduRatedFrequency for unit nameplate information.""",
}, # column
"inletRatedVA" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.3.1.9",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The inlet VA (VoltAmps) rating.
Deprecated: use pduRatedVA for unit nameplate information.""",
}, # column
"inletDeviceCapabilities" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.3.1.10",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Bits",
"rmsCurrent" : {
"nodetype" : "namednumber",
"number" : "0"
},
"peakCurrent" : {
"nodetype" : "namednumber",
"number" : "1"
},
"unbalancedCurrent" : {
"nodetype" : "namednumber",
"number" : "2"
},
"rmsVoltage" : {
"nodetype" : "namednumber",
"number" : "3"
},
"activePower" : {
"nodetype" : "namednumber",
"number" : "4"
},
"apparentPower" : {
"nodetype" : "namednumber",
"number" : "5"
},
"powerFactor" : {
"nodetype" : "namednumber",
"number" : "6"
},
"activeEnergy" : {
"nodetype" : "namednumber",
"number" : "7"
},
"apparentEnergy" : {
"nodetype" : "namednumber",
"number" : "8"
},
"surgeProtectorStatus" : {
"nodetype" : "namednumber",
"number" : "21"
},
"frequency" : {
"nodetype" : "namednumber",
"number" : "22"
},
"phaseAngle" : {
"nodetype" : "namednumber",
"number" : "23"
},
"residualCurrent" : {
"nodetype" : "namednumber",
"number" : "25"
},
"rcmState" : {
"nodetype" : "namednumber",
"number" : "26"
},
"reactivePower" : {
"nodetype" : "namednumber",
"number" : "28"
},
"powerQuality" : {
"nodetype" : "namednumber",
"number" : "31"
},
},
},
"access" : "readonly",
"description" :
"""A bit string which indicates which inlet sensors are available.""",
}, # column
"inletPoleCapabilities" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.3.1.11",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Bits",
"rmsCurrent" : {
"nodetype" : "namednumber",
"number" : "0"
},
"peakCurrent" : {
"nodetype" : "namednumber",
"number" : "1"
},
"rmsVoltage" : {
"nodetype" : "namednumber",
"number" : "3"
},
"activePower" : {
"nodetype" : "namednumber",
"number" : "4"
},
"apparentPower" : {
"nodetype" : "namednumber",
"number" : "5"
},
"powerFactor" : {
"nodetype" : "namednumber",
"number" : "6"
},
"activeEnergy" : {
"nodetype" : "namednumber",
"number" : "7"
},
"apparentEnergy" : {
"nodetype" : "namednumber",
"number" : "8"
},
"phaseAngle" : {
"nodetype" : "namednumber",
"number" : "23"
},
"rmsVoltageLN" : {
"nodetype" : "namednumber",
"number" : "24"
},
"reactivePower" : {
"nodetype" : "namednumber",
"number" : "28"
},
"displacementPowerFactor" : {
"nodetype" : "namednumber",
"number" : "34"
},
},
},
"access" : "readonly",
"description" :
"""A bit string which indicates which inletpole sensors are available.""",
}, # column
"inletPlugDescriptor" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.3.1.12",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""Description of the Plug""",
}, # column
"inletEnableState" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.3.1.13",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readwrite",
"description" :
"""Is this PDU operation is enabled for this inlet?
When PDU operation is disabled the sensors for this inlet and all
children will no longer be updated, and outlet switching is no longer
allowed. This is only meaningful for multi-inlet units if one inlet
is temporarily expected to be powered down.
Disabling the inlet of a single-inlet unit is forbidden and any
attempt to do so will result in an error.""",
}, # column
"inletRCMResidualOperatingCurrent" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.3.1.14",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The Residual Operating Current (Threshold) for the RCM State sensor.
For inlets without an RCM, any attempt to access this OID will return
NoSuchInstance error. The value of this OID is in milliamps.""",
}, # column
"inletSensorConfigurationTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.4",
"status" : "current",
"description" :
"""A list of configuration entries for an inlet sensor.""",
}, # table
"inletSensorConfigurationEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.4.1",
"status" : "current",
"linkage" : [
"pduId",
"inletId",
"sensorType",
],
"description" :
"""An entry containing objects for configuring an inlet sensor.""",
}, # row
"inletSensorLogAvailable" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.4.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readwrite",
"description" :
"""Is logging enabled for the sensor?""",
}, # column
"inletSensorUnits" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.4.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorUnitsEnumeration"},
},
"access" : "readonly",
"description" :
"""The base units.
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"inletSensorDecimalDigits" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.4.1.7",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The number of digits displayed to the right of the decimal point.
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"inletSensorAccuracy" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.4.1.8",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "HundredthsOfAPercentage"},
},
"access" : "readonly",
"description" :
"""The accuracy: how close (in percent) the measurement is to the actual value.
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"inletSensorResolution" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.4.1.9",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The resolution: the minimum difference between any two measured values
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"inletSensorTolerance" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.4.1.10",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The resolution: the difference between a sensor value and the actual value
The value of this OID variable should be scaled by
(inletSensorDecimalDigits + 1). For example, if the value is 50 and
inletSensorDecimalDigits is 2, then actual value is 0.05.
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"inletSensorMaximum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.4.1.11",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The largest possible reading as an unsigned integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled maximum exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"inletSensorMinimum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.4.1.12",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The smallest possible reading as an unsigned integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"inletSensorHysteresis" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.4.1.13",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The hysteresis used for deassertions
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"inletSensorStateChangeDelay" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.4.1.14",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The delay measured in samples before a state is asserted.
If the value is zero, then the state is asserted as soon as
it is detected; if it is non-zero, say n, then the assertion
condition must exist for n+1 consecutive samples before the corresponding
assertion event is reported.
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"inletSensorLowerCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.4.1.21",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The lower critical threshold as an unsigned integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"inletSensorLowerWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.4.1.22",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The lower warning threshold as an unsigned integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"inletSensorUpperCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.4.1.23",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The upper critical threshold as an unsigned integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"inletSensorUpperWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.4.1.24",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The upper warning threshold as an unsigned integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"inletSensorEnabledThresholds" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.4.1.25",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Bits",
"lowerCritical" : {
"nodetype" : "namednumber",
"number" : "0"
},
"lowerWarning" : {
"nodetype" : "namednumber",
"number" : "1"
},
"upperWarning" : {
"nodetype" : "namednumber",
"number" : "2"
},
"upperCritical" : {
"nodetype" : "namednumber",
"number" : "3"
},
},
},
"access" : "readwrite",
"description" :
"""A bit string which indicates which thresholds are enabled.
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"inletSensorSignedMaximum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.4.1.26",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The largest possible reading as a signed integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"inletSensorSignedMinimum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.4.1.27",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The smallest possible reading as a signed integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
This value determines whether to use the signed or unsigned threshold
and value columns: If the minimum is below zero the sensor can have
negative readings and the signed columns should be used.
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"inletSensorSignedLowerCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.4.1.28",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The lower critical threshold as a signed integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"inletSensorSignedLowerWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.4.1.29",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The lower warning threshold as a signed integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"inletSensorSignedUpperCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.4.1.30",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The upper critical threshold as a signed integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"inletSensorSignedUpperWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.4.1.31",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The upper warning threshold as a signed integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"inletPoleConfigurationTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.5",
"status" : "current",
"description" :
"""A list of inlet pole configuration entries. The number of
entries is given by the value of inletPoleCount for the PDU.""",
}, # table
"inletPoleConfigurationEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.5.1",
"status" : "current",
"linkage" : [
"pduId",
"inletId",
"inletPoleIndex",
],
"description" :
"""An entry containing parametersfor a particular inlet pole.""",
}, # row
"inletPoleLine" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.5.1.1",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "LineEnumeration"},
},
"access" : "readonly",
"description" :
"""The Phase for this inlet Pole.""",
}, # column
"inletPoleNode" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.5.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The node to which this inlet pole is connected""",
}, # column
"inletPoleSensorConfigurationTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.6",
"status" : "current",
"description" :
"""A list of configuration entries for an inlet pole sensor.""",
}, # table
"inletPoleSensorConfigurationEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.6.1",
"status" : "current",
"linkage" : [
"pduId",
"inletId",
"inletPoleIndex",
"sensorType",
],
"description" :
"""An entry containing objects
for configuring an inlet pole sensor.""",
}, # row
"inletPoleIndex" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.6.1.1",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "256"
},
],
"range" : {
"min" : "1",
"max" : "256"
},
},
},
"access" : "noaccess",
"description" :
"""A unique value for each inlet Pole. Its value
ranges between 1 and the value of inletPoleCount.""",
}, # column
"inletPoleSensorLogAvailable" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.6.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readwrite",
"description" :
"""Is logging enabled for the sensor?""",
}, # column
"inletPoleSensorUnits" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.6.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorUnitsEnumeration"},
},
"access" : "readonly",
"description" :
"""The base units.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"inletPoleSensorDecimalDigits" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.6.1.7",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The number of digits displayed to the right of the decimal point.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"inletPoleSensorAccuracy" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.6.1.8",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "HundredthsOfAPercentage"},
},
"access" : "readonly",
"description" :
"""The accuracy: how close (in percent) the measurement is to the actual value.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"inletPoleSensorResolution" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.6.1.9",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The resolution: the minimum difference between any two measured values
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"inletPoleSensorTolerance" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.6.1.10",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The resolution: the difference between a sensor value and the actual value
The value of this OID variable should be scaled by
(inletPoleSensorDecimalDigits + 1). For example, if the value is 50 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.05.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"inletPoleSensorMaximum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.6.1.11",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The largest possible reading as an unsigned integer.
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled maximum exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"inletPoleSensorMinimum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.6.1.12",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The smallest possible reading as an unsigned integer.
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"inletPoleSensorHysteresis" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.6.1.13",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The hysteresis used for deassertions
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"inletPoleSensorStateChangeDelay" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.6.1.14",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The delay measured in samples before a state is asserted.
If the value is zero, then the state is asserted as soon as
it is detected; if it is non-zero, say n, then the assertion
condition must exist for n+1 consecutive samples before the corresponding
assertion event is reported.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"inletPoleSensorLowerCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.6.1.21",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The lower critical threshold as an unsigned integer.
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"inletPoleSensorLowerWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.6.1.22",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The lower warning threshold as an unsigned integer.
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"inletPoleSensorUpperCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.6.1.23",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The upper critical threshold as an unsigned integer.
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"inletPoleSensorUpperWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.6.1.24",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The upper warning threshold as an unsigned integer.
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"inletPoleSensorEnabledThresholds" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.6.1.25",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Bits",
"lowerCritical" : {
"nodetype" : "namednumber",
"number" : "0"
},
"lowerWarning" : {
"nodetype" : "namednumber",
"number" : "1"
},
"upperWarning" : {
"nodetype" : "namednumber",
"number" : "2"
},
"upperCritical" : {
"nodetype" : "namednumber",
"number" : "3"
},
},
},
"access" : "readwrite",
"description" :
"""A bit string which indicates which thresholds are enabled.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"inletPoleSensorSignedMaximum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.6.1.26",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The largest possible reading as a signed integer.
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading.""",
}, # column
"inletPoleSensorSignedMinimum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.6.1.27",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The smallest possible reading as a signed integer.
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
This value determines whether to use the signed or unsigned threshold
and value columns: If the minimum is below zero the sensor can have
negative readings and the signed columns should be used.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"inletPoleSensorSignedLowerCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.6.1.28",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The lower critical threshold as a signed integer.
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading.""",
}, # column
"inletPoleSensorSignedLowerWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.6.1.29",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The lower warning threshold as a signed integer.
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading.""",
}, # column
"inletPoleSensorSignedUpperCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.6.1.30",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The upper critical threshold as a signed integer.
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading.""",
}, # column
"inletPoleSensorSignedUpperWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.3.6.1.31",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The upper warning threshold as a signed integer.
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading.""",
}, # column
"overCurrentProtector" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4",
}, # node
"overCurrentProtectorConfigurationTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.3",
"status" : "current",
"description" :
"""A list of overCurrentProtector configuration entries. The number of
entries is given by the value of overCurrentProtectorCount for the PDU.""",
}, # table
"overCurrentProtectorConfigurationEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.3.1",
"status" : "current",
"linkage" : [
"pduId",
"overCurrentProtectorIndex",
],
"description" :
"""An entry containing objects for a particular overCurrentProtector.""",
}, # row
"overCurrentProtectorIndex" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.3.1.1",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "256"
},
],
"range" : {
"min" : "1",
"max" : "256"
},
},
},
"access" : "noaccess",
"description" :
"""A unique value for each overcurrent protector. Its value
ranges between 1 and the value of overCurrentProtectorCount.""",
}, # column
"overCurrentProtectorLabel" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.3.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The label on the PDU identifying the overcurrent protector.""",
}, # column
"overCurrentProtectorName" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.3.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readwrite",
"description" :
"""The user-defined name.""",
}, # column
"overCurrentProtectorType" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.3.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "OverCurrentProtectorTypeEnumeration"},
},
"access" : "readonly",
"description" :
"""The type of overcurrent protector.""",
}, # column
"overCurrentProtectorRatedCurrent" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.3.1.5",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The current rating.""",
}, # column
"overCurrentProtectorPoleCount" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.3.1.6",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "3"
},
],
"range" : {
"min" : "1",
"max" : "3"
},
},
},
"access" : "readonly",
"description" :
"""The number of overCurrentProtector poles. """,
}, # column
"overCurrentProtectorCapabilities" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.3.1.9",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Bits",
"rmsCurrent" : {
"nodetype" : "namednumber",
"number" : "0"
},
"peakCurrent" : {
"nodetype" : "namednumber",
"number" : "1"
},
"trip" : {
"nodetype" : "namednumber",
"number" : "14"
},
},
},
"access" : "readonly",
"description" :
"""A bit string which indicates which overcurrent protector sensors are available.""",
}, # column
"overCurrentProtectorPowerSource" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.3.1.10",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "RowPointer"},
},
"access" : "readonly",
"description" :
"""This object allows discovery of how the PDU is wired. It indicates the
overCurrentProtector's power source which can one of the following:
overCurrentProtector
transfer switch
inlet
If the power source is an OverCurrentProtector, it
contains the OID of an overCurrentProtectorLabel in the
overCurrentProtectorConfiguration Table. The index of the OID can then be
used to reference other objects in the overCurrentProtectorConfigurationTable.
If the power source is an inlet, it contains the OID of
an inletLabel in the inletConfigurationTable. The index of the OID can
then be used to reference other objects in the inletConfigurationTable.
If the power source is a transferSwitch, it
contains the OID of a transferSwitchLabel in the
transferSwitchConfiguration Table. The index of the OID can then be
used to reference other objects in the transferSwitchConfigurationTable. """,
}, # column
"overCurrentProtectorSensorConfigurationTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.4",
"status" : "current",
"description" :
"""A list of overCurrentProtectorSensor configuration entries.""",
}, # table
"overCurrentProtectorSensorConfigurationEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.4.1",
"status" : "current",
"linkage" : [
"pduId",
"overCurrentProtectorIndex",
"sensorType",
],
"description" :
"""An overCurrentProtectorSensor entry containing objects for a
particular overCurrentProtector Sensor.""",
}, # row
"overCurrentProtectorSensorLogAvailable" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.4.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readwrite",
"description" :
"""Is logging available for this sensor?""",
}, # column
"overCurrentProtectorSensorUnits" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.4.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorUnitsEnumeration"},
},
"access" : "readonly",
"description" :
"""The base units
This parameter does not apply to sensors without numerical reading,
e.g. trip sensors.""",
}, # column
"overCurrentProtectorSensorDecimalDigits" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.4.1.7",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The number of digits displayed to the right of the decimal point.
This parameter does not apply to sensors without numerical reading,
e.g. trip sensors.""",
}, # column
"overCurrentProtectorSensorAccuracy" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.4.1.8",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "HundredthsOfAPercentage"},
},
"access" : "readonly",
"description" :
"""The accuracy: how close (in percent) the measurement is to the actual value
This parameter does not apply to sensors without numerical reading,
e.g. trip sensors.""",
}, # column
"overCurrentProtectorSensorResolution" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.4.1.9",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The resolution: the minimum difference between any two measured values
The value of this OID variable should be scaled by
overCurrentProtectorSensorDecimalDigits. For example, if the value is 1 and
overCurrentProtectorSensorDecimalDigits is 2, then actual value is 0.01.
This parameter does not apply to sensors without numerical reading,
e.g. trip sensors.""",
}, # column
"overCurrentProtectorSensorTolerance" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.4.1.10",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The resolution: the difference between a sensor value and the actual value
The value of this OID variable should be scaled by
(overCurrentProtectorSensorDecimalDigits + 1). For example, if the value is 50 and
overCurrentProtectorSensorDecimalDigits is 2, then actual value is 0.05.
This parameter does not apply to sensors without numerical reading,
e.g. trip sensors.""",
}, # column
"overCurrentProtectorSensorMaximum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.4.1.11",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The largest possible reading as an unsigned integer.
The value of this OID variable should be scaled by
overCurrentProtectorSensorDecimalDigits. For example, if the value is 1 and
overCurrentProtectorSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled maximum exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading,
e.g. trip sensors.""",
}, # column
"overCurrentProtectorSensorMinimum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.4.1.12",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The smallest possible reading as an unsigned integer.
The value of this OID variable should be scaled by
overCurrentProtectorSensorDecimalDigits. For example, if the value is 1 and
overCurrentProtectorSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading,
e.g. trip sensors.""",
}, # column
"overCurrentProtectorSensorHysteresis" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.4.1.13",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The hysteresis used for deassertions
The value of this OID variable should be scaled by
overCurrentProtectorSensorDecimalDigits. For example, if the value is 1 and
overCurrentProtectorSensorDecimalDigits is 2, then actual value is 0.01.
This parameter does not apply to sensors without numerical reading,
e.g. trip sensors.""",
}, # column
"overCurrentProtectorSensorStateChangeDelay" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.4.1.14",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The delay measured in samples before a state is asserted.
If the value is zero, then the state is asserted as soon as
it is detected; if it is non-zero, say n, then the assertion
condition must exist for n+1 consecutive samples before the corresponding
assertion event is reported.
This parameter does not apply to sensors without numerical reading,
e.g. trip sensors.""",
}, # column
"overCurrentProtectorSensorLowerCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.4.1.21",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The lower critical threshold as an unsigned integer.
The value of this OID variable should be scaled by
overCurrentProtectorSensorDecimalDigits. For example, if the value is 1 and
overCurrentProtectorSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading,
e.g. trip sensors.""",
}, # column
"overCurrentProtectorSensorLowerWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.4.1.22",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The lower warning threshold as an unsigned integer.
The value of this OID variable should be scaled by
overCurrentProtectorSensorDecimalDigits. For example, if the value is 1 and
overCurrentProtectorSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading,
e.g. trip sensors.""",
}, # column
"overCurrentProtectorSensorUpperCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.4.1.23",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The upper critical threshold as an unsigned integer.
The value of this OID variable should be scaled by
overCurrentProtectorSensorDecimalDigits. For example, if the value is 1 and
overCurrentProtectorSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading,
e.g. trip sensors.""",
}, # column
"overCurrentProtectorSensorUpperWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.4.1.24",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The upper warning threshold as an unsigned integer.
The value of this OID variable should be scaled by
overCurrentProtectorSensorDecimalDigits. For example, if the value is 1 and
overCurrentProtectorSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading,
e.g. trip sensors.""",
}, # column
"overCurrentProtectorSensorEnabledThresholds" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.4.1.25",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Bits",
"lowerCritical" : {
"nodetype" : "namednumber",
"number" : "0"
},
"lowerWarning" : {
"nodetype" : "namednumber",
"number" : "1"
},
"upperWarning" : {
"nodetype" : "namednumber",
"number" : "2"
},
"upperCritical" : {
"nodetype" : "namednumber",
"number" : "3"
},
},
},
"access" : "readwrite",
"description" :
"""A bit string which indicates which thresholds are enabled.
This parameter does not apply to sensors without numerical reading,
e.g. trip sensors.""",
}, # column
"overCurrentProtectorSensorSignedMaximum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.4.1.26",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The largest possible reading as a signed integer.
The value of this OID variable should be scaled by
overCurrentProtectorSensorDecimalDigits. For example, if the value is 1 and
overCurrentProtectorSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading,
e.g. trip sensors.""",
}, # column
"overCurrentProtectorSensorSignedMinimum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.4.1.27",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The smallest possible reading as a signed integer.
The value of this OID variable should be scaled by
overCurrentProtectorSensorDecimalDigits. For example, if the value is 1 and
overCurrentProtectorSensorDecimalDigits is 2, then actual value is 0.01.
This value determines whether to use the signed or unsigned threshold
and value columns: If the minimum is below zero the sensor can have
negative readings and the signed columns should be used.
This parameter does not apply to sensors without numerical reading,
e.g. trip sensors.""",
}, # column
"overCurrentProtectorSensorSignedLowerCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.4.1.28",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The lower critical threshold as a signed integer.
The value of this OID variable should be scaled by
overCurrentProtectorSensorDecimalDigits. For example, if the value is 1 and
overCurrentProtectorSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading,
e.g. trip sensors.""",
}, # column
"overCurrentProtectorSensorSignedLowerWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.4.1.29",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The lower warning threshold as a signed integer.
The value of this OID variable should be scaled by
overCurrentProtectorSensorDecimalDigits. For example, if the value is 1 and
overCurrentProtectorSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading,
e.g. trip sensors.""",
}, # column
"overCurrentProtectorSensorSignedUpperCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.4.1.30",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The upper critical threshold as a signed integer.
The value of this OID variable should be scaled by
overCurrentProtectorSensorDecimalDigits. For example, if the value is 1 and
overCurrentProtectorSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading,
e.g. trip sensors.""",
}, # column
"overCurrentProtectorSensorSignedUpperWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.4.1.31",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The upper warning threshold as a signed integer.
The value of this OID variable should be scaled by
overCurrentProtectorSensorDecimalDigits. For example, if the value is 1 and
overCurrentProtectorSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading,
e.g. trip sensors.""",
}, # column
"overCurrentProtectorPoleConfigurationTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.5",
"status" : "current",
"description" :
"""A list of overCurrentProtector pole configuration entries. The number of
entries is given by the value of overCurrentProtectorPoleCount for the PDU.""",
}, # table
"overCurrentProtectorPoleConfigurationEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.5.1",
"status" : "current",
"linkage" : [
"pduId",
"overCurrentProtectorIndex",
"overCurrentProtectorPoleIndex",
],
"description" :
"""An entry containing parametersfor a particular overCurrentProtector pole.""",
}, # row
"overCurrentProtectorPoleIndex" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.5.1.1",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "256"
},
],
"range" : {
"min" : "1",
"max" : "256"
},
},
},
"access" : "noaccess",
"description" :
"""A unique value for each overCurrentProtector Pole. Its value
ranges between 1 and the value of overCurrentProtectorPoleCount.""",
}, # column
"overCurrentProtectorPoleLine" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.5.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "LineEnumeration"},
},
"access" : "readonly",
"description" :
"""The Phase for this overCurrentProtector Pole.""",
}, # column
"overCurrentProtectorPoleInNode" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.5.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The node to which this overCurrentProtector pole input is connected""",
}, # column
"overCurrentProtectorPoleOutNode" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.4.5.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The node to which this overCurrentProtector pole output is connected""",
}, # column
"outlets" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5",
}, # node
"outletConfigurationTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.3",
"status" : "current",
"description" :
"""A list of outlet configuration entries. The number of
entries is given by the value of outletCount for the PDU.""",
}, # table
"outletConfigurationEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.3.1",
"status" : "current",
"linkage" : [
"pduId",
"outletId",
],
"description" :
"""An outlet entry containing parameters for a particular outlet.""",
}, # row
"outletId" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.3.1.1",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "256"
},
],
"range" : {
"min" : "1",
"max" : "256"
},
},
},
"access" : "noaccess",
"description" :
"""A unique value for each outlet. Its value
ranges between 1 and the value of outletCount.""",
}, # column
"outletLabel" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.3.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The label on the PDU identifying the outlet.""",
}, # column
"outletName" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.3.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readwrite",
"description" :
"""The user-defined name.""",
}, # column
"outletReceptacle" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.3.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "ReceptacleTypeEnumeration"},
},
"access" : "readonly",
"description" :
"""The plug.""",
}, # column
"outletPoleCount" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.3.1.5",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "2",
"max" : "4"
},
],
"range" : {
"min" : "2",
"max" : "4"
},
},
},
"access" : "readonly",
"description" :
"""The number of poles.""",
}, # column
"outletRatedVoltage" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.3.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The outlet voltage rating.""",
}, # column
"outletRatedCurrent" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.3.1.7",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The outlet current rating.""",
}, # column
"outletRatedVA" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.3.1.8",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The outlet VA (VoltAmps) rating.""",
}, # column
"outletDeviceCapabilities" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.3.1.10",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Bits",
"rmsCurrent" : {
"nodetype" : "namednumber",
"number" : "0"
},
"peakCurrent" : {
"nodetype" : "namednumber",
"number" : "1"
},
"unbalancedCurrent" : {
"nodetype" : "namednumber",
"number" : "2"
},
"rmsVoltage" : {
"nodetype" : "namednumber",
"number" : "3"
},
"activePower" : {
"nodetype" : "namednumber",
"number" : "4"
},
"apparentPower" : {
"nodetype" : "namednumber",
"number" : "5"
},
"powerFactor" : {
"nodetype" : "namednumber",
"number" : "6"
},
"activeEnergy" : {
"nodetype" : "namednumber",
"number" : "7"
},
"apparentEnergy" : {
"nodetype" : "namednumber",
"number" : "8"
},
"onOff" : {
"nodetype" : "namednumber",
"number" : "13"
},
"frequency" : {
"nodetype" : "namednumber",
"number" : "22"
},
"phaseAngle" : {
"nodetype" : "namednumber",
"number" : "23"
},
},
},
"access" : "readonly",
"description" :
"""A bit string which indicates which outlet sensors are available.""",
}, # column
"outletPoleCapabilities" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.3.1.11",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Bits",
"rmsCurrent" : {
"nodetype" : "namednumber",
"number" : "0"
},
"peakCurrent" : {
"nodetype" : "namednumber",
"number" : "1"
},
"rmsVoltage" : {
"nodetype" : "namednumber",
"number" : "3"
},
"activePower" : {
"nodetype" : "namednumber",
"number" : "4"
},
"apparentPower" : {
"nodetype" : "namednumber",
"number" : "5"
},
"powerFactor" : {
"nodetype" : "namednumber",
"number" : "6"
},
"activeEnergy" : {
"nodetype" : "namednumber",
"number" : "7"
},
"apparentEnergy" : {
"nodetype" : "namednumber",
"number" : "8"
},
"rmsVoltageLN" : {
"nodetype" : "namednumber",
"number" : "24"
},
},
},
"access" : "readonly",
"description" :
"""A bit string which indicates which outlet pole sensors are available.""",
}, # column
"outletPowerCyclingPowerOffPeriod" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.3.1.12",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The power-off period when an outlet is cycled;
overrides the global value;
specified in seconds;
0 <= value <= 3600 seconds.""",
}, # column
"outletStateOnStartup" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.3.1.13",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "OutletStateOnStartupEnumeration"},
},
"access" : "readwrite",
"description" :
"""The outlet state on device startup;
overrides the global value.
Note that this value is ignored if
relayBehaviorOnPowerLoss is set to latching.""",
}, # column
"outletUseGlobalPowerCyclingPowerOffPeriod" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.3.1.14",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readwrite",
"description" :
"""If this true, then use globalOutletPowerCyclingPowerOffPeriod.""",
}, # column
"outletSwitchable" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.3.1.28",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readonly",
"description" :
"""Is this outlet switchable?""",
}, # column
"outletReceptacleDescriptor" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.3.1.29",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""Description of the Receptacle""",
}, # column
"outletNonCritical" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.3.1.30",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readwrite",
"description" :
"""Is this outlet non-critical?""",
}, # column
"outletSequenceDelay" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.3.1.32",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The time interval between switching on this outlet and the next outlet in the
outlet sequence.
This applies to the following operations.
1) Switch all outlets on operation is executed
2) Power to the PDU is cycledThis applies only when all outlets are being switched on or cycled.
It is specified in seconds;
1 <= value <= 3600 seconds.
The actual time interval used can never be less than the inrushGuardDelay defined
in the unitConfigurationTable.
Examples:
1) inrush Guard Delay is 2 seconds and outletSequenceDelay is 5 seconds.
The time interval will be 5 seconds
2) inrush Guard Delay is 5 seconds and outletSequenceDelay is 2 seconds.
The time interval will be 5 seconds""",
}, # column
"outletPowerSource" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.3.1.33",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "RowPointer"},
},
"access" : "readonly",
"description" :
"""This object allows discovery of how the PDU is wired. It indicates the
outlet's power source which can one of the following:
overCurrentProtector
transfer switch
inlet
If the power source is an OverCurrentProtector, it
contains the OID of an overCurrentProtectorLabel in the
overCurrentProtectorConfiguration Table. The index of the OID can then be
used to reference other objects in the overCurrentProtectorConfigurationTable.
If the power source is an inlet, it contains the OID of
an inletLabel in the inletConfigurationTable. The index of the OID can
then be used to reference other objects in the inletConfigurationTable.
If the power source is a transferSwitch, it
contains the OID of a transferSwitchLabel in the
transferSwitchConfiguration Table. The index of the OID can then be
used to reference other objects in the transferSwitchConfigurationTable.""",
}, # column
"outletSensorConfigurationTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.4",
"status" : "current",
"description" :
"""A list of outlet configuration entries. The number of
entries is given by the value of outletCount for the PDU.""",
}, # table
"outletSensorConfigurationEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.4.1",
"status" : "current",
"linkage" : [
"pduId",
"outletId",
"sensorType",
],
"description" :
"""An entry containing parameters for an outlet sensor.""",
}, # row
"outletSensorLogAvailable" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.4.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readwrite",
"description" :
"""Is logging available for this sensor?""",
}, # column
"outletSensorUnits" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.4.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorUnitsEnumeration"},
},
"access" : "readonly",
"description" :
"""The base units.
This parameter does not apply to sensors without numerical reading,
e.g. outlet state sensors.""",
}, # column
"outletSensorDecimalDigits" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.4.1.7",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The number of digits displayed to the right of the decimal point
This parameter does not apply to sensors without numerical reading,
e.g. outlet state sensors.""",
}, # column
"outletSensorAccuracy" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.4.1.8",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "HundredthsOfAPercentage"},
},
"access" : "readonly",
"description" :
"""The accuracy: how close (in percent) the measurement is to the actual value
This parameter does not apply to sensors without numerical reading,
e.g. outlet state sensors.""",
}, # column
"outletSensorResolution" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.4.1.9",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The resolution: the minimum difference between any two measured values
The value of this OID variable should be scaled by
outletSensorDecimalDigits. For example, if the value is 1 and
outletSensorDecimalDigits is 2, then actual value is 0.01.
This parameter does not apply to sensors without numerical reading,
e.g. outlet state sensors.""",
}, # column
"outletSensorTolerance" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.4.1.10",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The resolution: the difference between a sensor value and the actual value
The value of this OID variable should be scaled by
(outletSensorDecimalDigits + 1). For example, if the value is 50 and
outletSensorDecimalDigits is 2, then actual value is 0.05.
This parameter does not apply to sensors without numerical reading,
e.g. outlet state sensors.""",
}, # column
"outletSensorMaximum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.4.1.11",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The largest possible reading as an unsigned integer.
The value of this OID variable should be scaled by
outletSensorDecimalDigits. For example, if the value is 1 and
outletSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled maximum exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading,
e.g. outlet state sensors.""",
}, # column
"outletSensorMinimum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.4.1.12",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The smallest possible reading as an unsigned integer.
The value of this OID variable should be scaled by
outletSensorDecimalDigits. For example, if the value is 1 and
outletSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading,
e.g. outlet state sensors.""",
}, # column
"outletSensorHysteresis" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.4.1.13",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The hysteresis used for deassertions
The value of this OID variable should be scaled by
outletSensorDecimalDigits. For example, if the value is 1 and
outletSensorDecimalDigits is 2, then actual value is 0.01.
This parameter does not apply to sensors without numerical reading,
e.g. outlet state sensors.""",
}, # column
"outletSensorStateChangeDelay" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.4.1.14",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The delay measured in samples before a state is asserted.
If the value is zero, then the state is asserted as soon as
it is detected; if it is non-zero, say n, then the assertion
condition must exist for n+1 consecutive samples before the corresponding
assertion event is reported.
This parameter does not apply to sensors without numerical reading,
e.g. outlet state sensors.""",
}, # column
"outletSensorLowerCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.4.1.21",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The lower critical threshold as an unsigned integer.
The value of this OID variable should be scaled by
outletSensorDecimalDigits. For example, if the value is 1 and
outletSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading,
e.g. outlet state sensors.""",
}, # column
"outletSensorLowerWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.4.1.22",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The lower warning threshold as an unsigned integer.
The value of this OID variable should be scaled by
outletSensorDecimalDigits. For example, if the value is 1 and
outletSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading,
e.g. outlet state sensors.""",
}, # column
"outletSensorUpperCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.4.1.23",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The upper critical threshold as an unsigned integer.
The value of this OID variable should be scaled by
outletSensorDecimalDigits. For example, if the value is 1 and
outletSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading,
e.g. outlet state sensors.""",
}, # column
"outletSensorUpperWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.4.1.24",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The upper warning threshold as an unsigned integer.
The value of this OID variable should be scaled by
outletSensorDecimalDigits. For example, if the value is 1 and
outletSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading,
e.g. outlet state sensors.""",
}, # column
"outletSensorEnabledThresholds" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.4.1.25",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Bits",
"lowerCritical" : {
"nodetype" : "namednumber",
"number" : "0"
},
"lowerWarning" : {
"nodetype" : "namednumber",
"number" : "1"
},
"upperWarning" : {
"nodetype" : "namednumber",
"number" : "2"
},
"upperCritical" : {
"nodetype" : "namednumber",
"number" : "3"
},
},
},
"access" : "readwrite",
"description" :
"""A bit string which indicates which thresholds are enabled.
This parameter does not apply to sensors without numerical reading,
e.g. outlet state sensors.""",
}, # column
"outletSensorSignedMaximum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.4.1.26",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The largest possible reading as a signed integer.
The value of this OID variable should be scaled by
outletSensorDecimalDigits. For example, if the value is 1 and
outletSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading,
e.g. outlet state sensors.""",
}, # column
"outletSensorSignedMinimum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.4.1.27",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The smallest possible reading as a signed integer.
The value of this OID variable should be scaled by
outletSensorDecimalDigits. For example, if the value is 1 and
outletSensorDecimalDigits is 2, then actual value is 0.01.
This value determines whether to use the signed or unsigned threshold
and value columns: If the minimum is below zero the sensor can have
negative readings and the signed columns should be used.
This parameter does not apply to sensors without numerical reading,
e.g. outlet state sensors.""",
}, # column
"outletSensorSignedLowerCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.4.1.28",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The lower critical threshold as a signed integer.
The value of this OID variable should be scaled by
outletSensorDecimalDigits. For example, if the value is 1 and
outletSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading,
e.g. outlet state sensors.""",
}, # column
"outletSensorSignedLowerWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.4.1.29",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The lower warning threshold as a signed integer.
The value of this OID variable should be scaled by
outletSensorDecimalDigits. For example, if the value is 1 and
outletSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading,
e.g. outlet state sensors.""",
}, # column
"outletSensorSignedUpperCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.4.1.30",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The upper critical threshold as a signed integer.
The value of this OID variable should be scaled by
outletSensorDecimalDigits. For example, if the value is 1 and
outletSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading,
e.g. outlet state sensors.""",
}, # column
"outletSensorSignedUpperWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.4.1.31",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The upper warning threshold as a signed integer.
The value of this OID variable should be scaled by
outletSensorDecimalDigits. For example, if the value is 1 and
outletSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading,
e.g. outlet state sensors.""",
}, # column
"outletPoleConfigurationTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.5",
"status" : "current",
"description" :
"""A list of outlet pole configuration entries. The number of
entries is given by the value of outletPoleCount for the PDU.""",
}, # table
"outletPoleConfigurationEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.5.1",
"status" : "current",
"linkage" : [
"pduId",
"outletId",
"outletPoleIndex",
],
"description" :
"""An entry containing parametersfor a particular outlet pole.""",
}, # row
"outletPoleLine" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.5.1.1",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "LineEnumeration"},
},
"access" : "readonly",
"description" :
"""The Phase for this inlet Pole.""",
}, # column
"outletPoleNode" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.5.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The node to which this outlet pole is connected""",
}, # column
"outletPoleSensorConfigurationTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.6",
"status" : "current",
"description" :
"""A list of outlet pole sensor configuration entries. The number of
entries is given by the value of outletPoleCount.""",
}, # table
"outletPoleSensorConfigurationEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.6.1",
"status" : "current",
"linkage" : [
"pduId",
"outletId",
"outletPoleIndex",
"sensorType",
],
"description" :
"""An entry containing objects
for configuring an outlet pole sensor.""",
}, # row
"outletPoleIndex" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.6.1.1",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "256"
},
],
"range" : {
"min" : "1",
"max" : "256"
},
},
},
"access" : "noaccess",
"description" :
"""A unique value for each outlet Pole. Its value
ranges between 1 and the value of outletPoleCount.""",
}, # column
"outletPoleSensorLogAvailable" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.6.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readwrite",
"description" :
"""Is logging enabled for the sensor?""",
}, # column
"outletPoleSensorUnits" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.6.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorUnitsEnumeration"},
},
"access" : "readonly",
"description" :
"""The base units.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"outletPoleSensorDecimalDigits" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.6.1.7",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The number of digits displayed to the right of the decimal point.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"outletPoleSensorAccuracy" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.6.1.8",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "HundredthsOfAPercentage"},
},
"access" : "readonly",
"description" :
"""The accuracy: how close (in percent) the measurement is to the actual value.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"outletPoleSensorResolution" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.6.1.9",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The resolution: the minimum difference between any two measured values
The value of this OID variable should be scaled by
outletPoleSensorDecimalDigits. For example, if the value is 50 and
outletPoleSensorDecimalDigits is 2, then actual value is 0.05.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"outletPoleSensorTolerance" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.6.1.10",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The resolution: the difference between a sensor value and the actual value
The value of this OID variable should be scaled by
(outletPoleSensorDecimalDigits + 1). For example, if the value is 50 and
outletPoleSensorDecimalDigits is 2, then actual value is 0.05.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"outletPoleSensorMaximum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.6.1.11",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The largest possible reading as an unsigned integer.
The value of this OID variable should be scaled by
outletPoleSensorDecimalDigits. For example, if the value is 1 and
outletPoleSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled maximum exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"outletPoleSensorMinimum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.6.1.12",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The smallest possible reading as an unsigned integer.
The value of this OID variable should be scaled by
outletPoleSensorDecimalDigits. For example, if the value is 1 and
outletPoleSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"outletPoleSensorHysteresis" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.6.1.13",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The hysteresis used for deassertions
The value of this OID variable should be scaled by
outletPoleSensorDecimalDigits. For example, if the value is 1 and
outletPoleSensorDecimalDigits is 2, then actual value is 0.01.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"outletPoleSensorStateChangeDelay" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.6.1.14",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The delay measured in samples before a state is asserted.
If the value is zero, then the state is asserted as soon as
it is detected; if it is non-zero, say n, then the assertion
condition must exist for n+1 consecutive samples before the corresponding
assertion event is reported.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"outletPoleSensorLowerCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.6.1.21",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The lower critical threshold as an unsigned integer.
The value of this OID variable should be scaled by
outletPoleSensorDecimalDigits. For example, if the value is 1 and
outletPoleSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"outletPoleSensorLowerWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.6.1.22",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The lower warning threshold as an unsigned integer.
The value of this OID variable should be scaled by
outletPoleSensorDecimalDigits. For example, if the value is 1 and
outletPoleSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"outletPoleSensorUpperCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.6.1.23",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The upper critical threshold as an unsigned integer.
The value of this OID variable should be scaled by
outletPoleSensorDecimalDigits. For example, if the value is 1 and
outletPoleSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"outletPoleSensorUpperWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.6.1.24",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The upper warning threshold as an unsigned integer.
The value of this OID variable should be scaled by
outletPoleSensorDecimalDigits. For example, if the value is 1 and
outletPoleSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"outletPoleSensorEnabledThresholds" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.6.1.25",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Bits",
"lowerCritical" : {
"nodetype" : "namednumber",
"number" : "0"
},
"lowerWarning" : {
"nodetype" : "namednumber",
"number" : "1"
},
"upperWarning" : {
"nodetype" : "namednumber",
"number" : "2"
},
"upperCritical" : {
"nodetype" : "namednumber",
"number" : "3"
},
},
},
"access" : "readwrite",
"description" :
"""A bit string which indicates which thresholds are enabled.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"outletPoleSensorSignedMaximum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.6.1.26",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The largest possible reading as a signed integer.
The value of this OID variable should be scaled by
outletPoleSensorDecimalDigits. For example, if the value is 1 and
outletPoleSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading.""",
}, # column
"outletPoleSensorSignedMinimum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.6.1.27",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The smallest possible reading as a signed integer.
The value of this OID variable should be scaled by
outletPoleSensorDecimalDigits. For example, if the value is 1 and
outletPoleSensorDecimalDigits is 2, then actual value is 0.01.
This value determines whether to use the signed or unsigned threshold
and value columns: If the minimum is below zero the sensor can have
negative readings and the signed columns should be used.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"outletPoleSensorSignedLowerCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.6.1.28",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The lower critical threshold as a signed integer.
The value of this OID variable should be scaled by
outletPoleSensorDecimalDigits. For example, if the value is 1 and
outletPoleSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading.""",
}, # column
"outletPoleSensorSignedLowerWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.6.1.29",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The lower warning threshold as a signed integer.
The value of this OID variable should be scaled by
outletPoleSensorDecimalDigits. For example, if the value is 1 and
outletPoleSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading.""",
}, # column
"outletPoleSensorSignedUpperCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.6.1.30",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The upper critical threshold as a signed integer.
The value of this OID variable should be scaled by
outletPoleSensorDecimalDigits. For example, if the value is 1 and
outletPoleSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading.""",
}, # column
"outletPoleSensorSignedUpperWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.5.6.1.31",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The upper warning threshold as a signed integer.
The value of this OID variable should be scaled by
outletPoleSensorDecimalDigits. For example, if the value is 1 and
outletPoleSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading.""",
}, # column
"externalSensors" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6",
}, # node
"externalSensorConfigurationTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.3",
"status" : "current",
"description" :
"""A list of externalSensor configuration entries. The number of
entries is given by the value of externalSensorCount for the PDU.""",
}, # table
"externalSensorConfigurationEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.3.1",
"status" : "current",
"linkage" : [
"pduId",
"sensorID",
],
"description" :
"""An entry containing parameters for an external sensor.""",
}, # row
"sensorID" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.3.1.1",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "256"
},
],
"range" : {
"min" : "1",
"max" : "256"
},
},
},
"access" : "noaccess",
"description" :
"""A unique value for each sensor. Its value
ranges between 1 and the value of externalSensorCount.""",
}, # column
"externalSensorType" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.3.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorTypeEnumeration"},
},
"access" : "readonly",
"description" :
"""The type of sensor.""",
}, # column
"externalSensorSerialNumber" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.3.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The sensor serial number.""",
}, # column
"externalSensorName" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.3.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readwrite",
"description" :
"""The user-defined name.""",
}, # column
"externalSensorDescription" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.3.1.5",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readwrite",
"description" :
"""The user-defined description.""",
}, # column
"externalSensorXCoordinate" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.3.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readwrite",
"description" :
"""The X coordinate.""",
}, # column
"externalSensorYCoordinate" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.3.1.7",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readwrite",
"description" :
"""The Y coordinate.""",
}, # column
"externalSensorZCoordinate" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.3.1.8",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readwrite",
"description" :
"""The Z coordinate.""",
}, # column
"externalSensorChannelNumber" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.3.1.9",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The channel number, applies only to contact sensors;
-1 for other sensors""",
}, # column
"externalOnOffSensorSubtype" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.3.1.10",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorTypeEnumeration"},
},
"access" : "readwrite",
"description" :
"""The subtype of the binary sensor""",
}, # column
"externalSensorLogAvailable" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.3.1.14",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readwrite",
"description" :
"""Is logging available for this sensor?""",
}, # column
"externalSensorUnits" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.3.1.16",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorUnitsEnumeration"},
},
"access" : "readonly",
"description" :
"""The base units
This parameter does not apply
to onOff, trip, vibration, waterDetection,
smokeDetection sensors.
""",
}, # column
"externalSensorDecimalDigits" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.3.1.17",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The number of digits displayed to the right of the decimal point
This parameter does not apply
to onOff, trip, vibration, waterDetection,
smokeDetection sensors.""",
}, # column
"externalSensorAccuracy" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.3.1.18",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "HundredthsOfAPercentage"},
},
"access" : "readonly",
"description" :
"""The accuracy: how close (in percent) the measurement is to the actual value
This parameter does not apply
to onOff, trip, vibration, waterDetection,
smokeDetection sensors.""",
}, # column
"externalSensorResolution" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.3.1.19",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The resolution: the minimum difference between any two measured values
The value of this OID variable should be scaled by
externalSensorDecimalDigits. For example, if the value is 1 and
externalSensorDecimalDigits is 2, then actual value is 0.01.
This parameter does not apply
to onOff, trip, vibration, waterDetection,
smokeDetection sensors.""",
}, # column
"externalSensorTolerance" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.3.1.20",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The resolution: the difference between a sensor value and the actual value
The value of this OID variable should be scaled by
(externalSensorDecimalDigits + 1). For example, if the value is 50 and
externalSensorDecimalDigits is 2, then actual value is 0.05.
This parameter does not apply
to onOff, trip, vibration, waterDetection,
smokeDetection sensors.""",
}, # column
"externalSensorMaximum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.3.1.21",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The largest possible value
The value of this OID variable should be scaled by
externalSensorDecimalDigits. For example, if the value is 1 and
externalSensorDecimalDigits is 2, then actual value is 0.01.
This parameter does not apply
to onOff, trip, vibration, waterDetection,
smokeDetection sensors.""",
}, # column
"externalSensorMinimum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.3.1.22",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The smallest possible value
The value of this OID variable should be scaled by
externalSensorDecimalDigits. For example, if the value is 1 and
externalSensorDecimalDigits is 2, then actual value is 0.01.
This parameter does not apply
to onOff, trip, vibration, waterDetection,
smokeDetection sensors.""",
}, # column
"externalSensorHysteresis" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.3.1.23",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The hysteresis used for deassertions
The value of this OID variable should be scaled by
externalSensorDecimalDigits. For example, if the value is 1 and
externalSensorDecimalDigits is 2, then actual value is 0.01.
This parameter does not apply
to onOff, trip, vibration, waterDetection,
smokeDetection sensors.""",
}, # column
"externalSensorStateChangeDelay" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.3.1.24",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The delay measured in samples before a state is asserted.
If the value is zero, then the state is asserted as soon as
it is detected; if it is non-zero, say n, then the assertion
condition must exist for n+1 consecutive samples before the corresponding
assertion event is reported.
At present, this value cannot be written (set)""",
}, # column
"externalSensorLowerCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.3.1.31",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The lower critical threshold
The value of this OID variable should be scaled by
externalSensorDecimalDigits. For example, if the value is 1 and
externalSensorDecimalDigits is 2, then actual value is 0.01.
This parameter does not apply
to onOff, trip, vibration, waterDetection,
smokeDetection sensors.""",
}, # column
"externalSensorLowerWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.3.1.32",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The lower non-critical (warning) threshold
The value of this OID variable should be scaled by
externalSensorDecimalDigits. For example, if the value is 1 and
externalSensorDecimalDigits is 2, then actual value is 0.01.
This parameter does not apply
to onOff, trip, vibration, waterDetection,
smokeDetection sensors.""",
}, # column
"externalSensorUpperCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.3.1.33",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The upper critical threshold
The value of this OID variable should be scaled by
externalSensorDecimalDigits. For example, if the value is 1 and
externalSensorDecimalDigits is 2, then actual value is 0.01.
This parameter does not apply
to onOff, trip, vibration, waterDetection,
smokeDetection sensors.""",
}, # column
"externalSensorUpperWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.3.1.34",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The upper non-critical (warning) threshold
The value of this OID variable should be scaled by
externalSensorDecimalDigits. For example, if the value is 1 and
externalSensorDecimalDigits is 2, then actual value is 0.01.
This parameter does not apply
to onOff, trip, vibration, waterDetection,
smokeDetection sensors.""",
}, # column
"externalSensorEnabledThresholds" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.3.1.35",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Bits",
"lowerCritical" : {
"nodetype" : "namednumber",
"number" : "0"
},
"lowerWarning" : {
"nodetype" : "namednumber",
"number" : "1"
},
"upperWarning" : {
"nodetype" : "namednumber",
"number" : "2"
},
"upperCritical" : {
"nodetype" : "namednumber",
"number" : "3"
},
},
},
"access" : "readwrite",
"description" :
"""A bit string which indicates which thresholds are enabled.
This parameter does not apply
to onOff, trip, vibration, waterDetection,
smokeDetection sensors """,
}, # column
"externalSensorIsActuator" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.3.1.36",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readonly",
"description" :
"""Is this an actuator?
True: It is an actuator
False: It is not an actuator""",
}, # column
"externalSensorPosition" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.3.1.37",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The position of the sensor.
The format is a semicolon separated list of Keyword:value pairs - Keyword1:Value1;Keyword2:value2;...
Keyword can be one of the following:
ONBOARD, DEVICE-1WIREPORT, HUBPORT, CHAIN-POSITION
Examples
1) Onboard Sensor
ONBOARD:CC1
2) Old sensor connected to device 1-wire port
DEVICE-1WIREPORT:2
3) New style sensor connected to device 1-wire port
DEVICE-1WIREPORT:2;CHAIN-POSITION:3
4) New style sensor connected to hub port 3
DEVICE-1WIREPORT:2;CHAIN-POSITION:1;HUBPORT:3;CHAIN-POSITION:1
5) Old style sensor connected to end of new style sensor chain
DEVICE-1WIREPORT:2;
""",
}, # column
"externalSensorUseDefaultThresholds" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.3.1.38",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readwrite",
"description" :
"""Use default thresholds for this sensor?
True: Use Default thresholds for this sensor
False: Do not Use Default thresholds for this sensor""",
}, # column
"externalSensorAlarmedToNormalDelay" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.3.1.39",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The delay in seconds for transitions from the alarmed state to the normal state.
If the sensor type is motionDetection,
then it can set to a value greater >= 0
For all other sensor types,
the value is 0 and cannot be set to any other value.""",
}, # column
"externalSensorTypeDefaultThresholdsTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.4",
"status" : "current",
"description" :
"""A list of entries listing the default thresholds for each sensor type. """,
}, # table
"externalSensorTypeDefaultThresholdsEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.4.1",
"status" : "current",
"linkage" : [
"pduId",
"sensorType",
],
"description" :
"""An entry containing default thresholds for a sensor type.""",
}, # row
"externalSensorTypeDefaultHysteresis" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.4.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The hysteresis used for deassertions
The value of this OID variable should be scaled by
externalSensorDecimalDigits. For example, if the value is 1 and
externalSensorDecimalDigits is 2, then actual value is 0.01.
This parameter does not apply
to onOff, trip, vibration, waterDetection,
smokeDetection sensors.""",
}, # column
"externalSensorTypeDefaultStateChangeDelay" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.4.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The delay measured in samples before a state is asserted.
If the value is zero, then the state is asserted as soon as
it is detected; if it is non-zero, say n, then the assertion
condition must exist for n+1 consecutive samples before the corresponding
assertion event is reported.
At present, this value cannot be written (set)""",
}, # column
"externalSensorTypeDefaultLowerCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.4.1.5",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The lower critical threshold
The value of this OID variable should be scaled by
externalSensorDecimalDigits. For example, if the value is 1 and
externalSensorDecimalDigits is 2, then actual value is 0.01.
This parameter does not apply
to onOff, trip, vibration, waterDetection,
smokeDetection sensors.""",
}, # column
"externalSensorTypeDefaultLowerWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.4.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The lower non-critical (warning) threshold
The value of this OID variable should be scaled by
externalSensorDecimalDigits. For example, if the value is 1 and
externalSensorDecimalDigits is 2, then actual value is 0.01.
This parameter does not apply
to onOff, trip, vibration, waterDetection,
smokeDetection sensors.""",
}, # column
"externalSensorTypeDefaultUpperCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.4.1.7",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The upper critical threshold
The value of this OID variable should be scaled by
externalSensorDecimalDigits. For example, if the value is 1 and
externalSensorDecimalDigits is 2, then actual value is 0.01.
This parameter does not apply
to onOff, trip, vibration, waterDetection,
smokeDetection sensors.""",
}, # column
"externalSensorTypeDefaultUpperWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.4.1.8",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The upper non-critical (warning) threshold
The value of this OID variable should be scaled by
externalSensorDecimalDigits. For example, if the value is 1 and
externalSensorDecimalDigits is 2, then actual value is 0.01.
This parameter does not apply
to onOff, trip, vibration, waterDetection,
smokeDetection sensors.""",
}, # column
"externalSensorTypeDefaultEnabledThresholds" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.4.1.9",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Bits",
"lowerCritical" : {
"nodetype" : "namednumber",
"number" : "0"
},
"lowerWarning" : {
"nodetype" : "namednumber",
"number" : "1"
},
"upperWarning" : {
"nodetype" : "namednumber",
"number" : "2"
},
"upperCritical" : {
"nodetype" : "namednumber",
"number" : "3"
},
},
},
"access" : "readwrite",
"description" :
"""A bit string which indicates which thresholds are enabled.
This parameter does not apply
to onOff, trip, vibration, waterDetection,
smokeDetection sensors """,
}, # column
"peripheralDevicePackageTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.5",
"status" : "current",
"description" :
"""A list of entries for the peripheral Device Packages connected to each PDU. The number of
entries is given by the value of peripheralDevicePackagesCount.""",
}, # table
"peripheralDevicePackageEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.5.1",
"status" : "current",
"linkage" : [
"pduId",
"peripheralDevicePackageId",
],
"description" :
"""An entry containing objects for a controller.""",
}, # row
"peripheralDevicePackageId" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.5.1.1",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "256"
},
],
"range" : {
"min" : "1",
"max" : "256"
},
},
},
"access" : "noaccess",
"description" :
"""A unique value for each peripheral device package.""",
}, # column
"peripheralDevicePackageSerialNumber" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.5.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The peripheral device package serial number.""",
}, # column
"peripheralDevicePackageModel" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.5.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The peripheral device package model.
Examples are DX-D2C6, DPX2-T1, DPX2-T1H1, DPX2-T2H1, DPX2-T3H1""",
}, # column
"peripheralDevicePackageFirmwareVersion" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.5.1.5",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The peripheral device package firmware version.""",
}, # column
"peripheralDevicePackageMinFirmwareVersion" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.5.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The peripheral device package minimum firmware version.
This field may be empty. If it is not empty, then it shall not be possible
to downgrade the peripheral device firmware to a
version < minimum firmware version number.""",
}, # column
"peripheralDevicePackageFirmwareTimeStamp" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.5.1.7",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The time when the peripheralDevicePackage firmware was updated in UNIX(epoch)time.
It is measured in seconds relative to January 1, 1970 (midnight UTC/GMT),
i.e a value of 0 indicates January 1, 1970 (midnight UTC/GMT).""",
}, # column
"peripheralDevicePackagePosition" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.5.1.8",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The position of the package.
The format is a semicolon separated list of Keyword:value pairs -
Keyword1:Value1;Keyword2:value2;...
Keyword can be one of the following:
ONBOARD, DEVICE-1WIREPORT, HUBPORT, CHAIN-POSITION
Examples
1) Onboard Sensor
ONBOARD:CC1
2) Old sensor connected to device 1-wire port
DEVICE-1WIREPORT:2
3) New style sensor connected to device 1-wire port
DEVICE-1WIREPORT:2;CHAIN-POSITION:3
4) New style sensor connected to hub port 3
DEVICE-1WIREPORT:2;CHAIN-POSITION:1;HUBPORT:3;CHAIN-POSITION:1
5) Old style sensor connected to end of new style sensor chain
DEVICE-1WIREPORT:2;
""",
}, # column
"peripheralDevicePackageState" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.6.5.1.9",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The state of the package.""",
}, # column
"serverReachability" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.7",
}, # node
"serverReachabilityTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.7.3",
"status" : "current",
"description" :
"""A list of server entries. The number of
entries is given by the value of serverCount for the PDU.""",
}, # table
"serverReachabilityEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.7.3.1",
"status" : "current",
"linkage" : [
"pduId",
"serverID",
],
"description" :
"""An entry containing parameters for a server.""",
}, # row
"serverID" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.7.3.1.1",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "256"
},
],
"range" : {
"min" : "1",
"max" : "256"
},
},
},
"access" : "noaccess",
"description" :
"""A unique value for each server. Its value
ranges between 1 and the value of serverCount for that PDU""",
}, # column
"serverIPAddress" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.7.3.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The IP Address/host name of the server""",
}, # column
"serverPingEnabled" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.7.3.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readwrite",
"description" :
"""Is ping enabled for this server?""",
}, # column
"wires" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.8",
}, # node
"wireConfigurationTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.8.3",
"status" : "deprecated",
"description" :
"""A list of wire configuration entries. The number of
entries is given by the value of wireCount for the PDU.""",
}, # table
"wireConfigurationEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.8.3.1",
"status" : "deprecated",
"linkage" : [
"pduId",
"wireId",
],
"description" :
"""An entry containing objects for a particular wire.""",
}, # row
"wireId" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.8.3.1.1",
"status" : "deprecated",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "256"
},
],
"range" : {
"min" : "1",
"max" : "256"
},
},
},
"access" : "noaccess",
"description" :
"""A unique value for each wire. Its value
ranges between 1 and the value of wireCount.""",
}, # column
"wireLabel" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.8.3.1.2",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The label on the PDU identifying the wire.""",
}, # column
"wireCapabilities" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.8.3.1.3",
"status" : "deprecated",
"syntax" : {
"type" : {
"basetype" : "Bits",
"rmsCurrent" : {
"nodetype" : "namednumber",
"number" : "0"
},
"peakCurrent" : {
"nodetype" : "namednumber",
"number" : "1"
},
"unbalancedCurrent" : {
"nodetype" : "namednumber",
"number" : "2"
},
"rmsVoltage" : {
"nodetype" : "namednumber",
"number" : "3"
},
"activePower" : {
"nodetype" : "namednumber",
"number" : "4"
},
"apparentPower" : {
"nodetype" : "namednumber",
"number" : "5"
},
"powerFactor" : {
"nodetype" : "namednumber",
"number" : "6"
},
"activeEnergy" : {
"nodetype" : "namednumber",
"number" : "7"
},
"apparentEnergy" : {
"nodetype" : "namednumber",
"number" : "8"
},
},
},
"access" : "readonly",
"description" :
"""A bit string which indicates which wire sensors are available.""",
}, # column
"wirePowerSource" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.8.3.1.4",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "RowPointer"},
},
"access" : "readonly",
"description" :
"""This object allows discovery of how the PDU is wired. It indicates the
wire's power source which can one of the following:
overCurrentProtector
transfer switch
inlet
If the power source is an OverCurrentProtector, it
contains the OID of an overCurrentProtectorLabel in the
overCurrentProtectorConfiguration Table. The index of the OID can then be
used to reference other objects in the overCurrentProtectorConfigurationTable.
If the power source is an inlet, it contains the OID of
an inletLabel in the inletConfigurationTable. The index of the OID can
then be used to reference other objects in the inletConfigurationTable.
If the power source is a transferSwitch, it
contains the OID of a transferSwitchLabel in the
transferSwitchConfiguration Table. The index of the OID can then be
used to reference other objects in the transferSwitchConfigurationTable. """,
}, # column
"wireSensorConfigurationTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.8.4",
"status" : "deprecated",
"description" :
"""A list of wireSensor configuration entries.""",
}, # table
"wireSensorConfigurationEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.8.4.1",
"status" : "deprecated",
"linkage" : [
"pduId",
"wireId",
"sensorType",
],
"description" :
"""An wireSensor entry containing objects for a
particular wire Sensor.""",
}, # row
"wireSensorLogAvailable" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.8.4.1.4",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readwrite",
"description" :
"""Is logging available for this sensor?""",
}, # column
"wireSensorUnits" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.8.4.1.6",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorUnitsEnumeration"},
},
"access" : "readonly",
"description" :
"""The base units""",
}, # column
"wireSensorDecimalDigits" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.8.4.1.7",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The number of digits displayed to the right of the decimal point.""",
}, # column
"wireSensorAccuracy" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.8.4.1.8",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "HundredthsOfAPercentage"},
},
"access" : "readonly",
"description" :
"""The accuracy: how close (in percent) the measurement is to the actual value""",
}, # column
"wireSensorResolution" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.8.4.1.9",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The resolution: the minimum difference between any two measured values
The value of this OID variable should be scaled by
wireSensorDecimalDigits. For example, if the value is 1 and
wireSensorDecimalDigits is 2, then actual value is 0.01.""",
}, # column
"wireSensorTolerance" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.8.4.1.10",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The resolution: the difference between a sensor value and the actual value
The value of this OID variable should be scaled by
(wireSensorDecimalDigits + 1). For example, if the value is 50 and
wireSensorDecimalDigits is 2, then actual value is 0.05.""",
}, # column
"wireSensorMaximum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.8.4.1.11",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The largest possible value
The value of this OID variable should be scaled by
wireSensorDecimalDigits. For example, if the value is 1 and
wireSensorDecimalDigits is 2, then actual value is 0.01.""",
}, # column
"wireSensorMinimum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.8.4.1.12",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The smallest possible value
The value of this OID variable should be scaled by
wireSensorDecimalDigits. For example, if the value is 1 and
wireSensorDecimalDigits is 2, then actual value is 0.01.""",
}, # column
"wireSensorHysteresis" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.8.4.1.13",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The hysteresis used for deassertions
The value of this OID variable should be scaled by
wireSensorDecimalDigits. For example, if the value is 1 and
wireSensorDecimalDigits is 2, then actual value is 0.01.""",
}, # column
"wireSensorStateChangeDelay" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.8.4.1.14",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The delay measured in samples before a state is asserted.
If the value is zero, then the state is asserted as soon as
it is detected; if it is non-zero, say n, then the assertion
condition must exist for n+1 consecutive samples before the corresponding
assertion event is reported.
At present, this value cannot be written (set)""",
}, # column
"wireSensorLowerCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.8.4.1.21",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The lower critical threshold
The value of this OID variable should be scaled by
wireSensorDecimalDigits. For example, if the value is 1 and
wireSensorDecimalDigits is 2, then actual value is 0.01.""",
}, # column
"wireSensorLowerWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.8.4.1.22",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The lower non-critical (warning) threshold
The value of this OID variable should be scaled by
wireSensorDecimalDigits. For example, if the value is 1 and
wireSensorDecimalDigits is 2, then actual value is 0.01.""",
}, # column
"wireSensorUpperCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.8.4.1.23",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The upper critical threshold
The value of this OID variable should be scaled by
wireSensorDecimalDigits. For example, if the value is 1 and
wireSensorDecimalDigits is 2, then actual value is 0.01.""",
}, # column
"wireSensorUpperWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.8.4.1.24",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The upper non-critical (warning) threshold
The value of this OID variable should be scaled by
wireSensorDecimalDigits. For example, if the value is 1 and
wireSensorDecimalDigits is 2, then actual value is 0.01.""",
}, # column
"wireSensorEnabledThresholds" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.8.4.1.25",
"status" : "deprecated",
"syntax" : {
"type" : {
"basetype" : "Bits",
"lowerCritical" : {
"nodetype" : "namednumber",
"number" : "0"
},
"lowerWarning" : {
"nodetype" : "namednumber",
"number" : "1"
},
"upperWarning" : {
"nodetype" : "namednumber",
"number" : "2"
},
"upperCritical" : {
"nodetype" : "namednumber",
"number" : "3"
},
},
},
"access" : "readwrite",
"description" :
"""A bit string which indicates which thresholds are enabled.""",
}, # column
"transferSwitch" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9",
}, # node
"transferSwitchConfigurationTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.3",
"status" : "current",
"description" :
"""A list of transfer switch configuration entries. The number of
entries is given by the value of transferSwitchCount.""",
}, # table
"transferSwitchConfigurationEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.3.1",
"status" : "current",
"linkage" : [
"pduId",
"transferSwitchId",
],
"description" :
"""An entry containing objects for a particular transferSwitch.""",
}, # row
"transferSwitchId" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.3.1.1",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "256"
},
],
"range" : {
"min" : "1",
"max" : "256"
},
},
},
"access" : "noaccess",
"description" :
"""A unique value for each transfer switch. Its value
ranges between 1 and the value of transferSwitchCount.""",
}, # column
"transferSwitchLabel" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.3.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""The label on the PDU identifying the TS.""",
}, # column
"transferSwitchName" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.3.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readwrite",
"description" :
"""The user-defined name of the transferSwitch.""",
}, # column
"transferSwitchPreferredInlet" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.3.1.4",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "64"
},
],
"range" : {
"min" : "1",
"max" : "64"
},
},
},
"access" : "readonly",
"description" :
"""The preferred Inlet. The TS powers the outlet from the preferred inlet when acceptable power
is available from the preferred inlet. """,
}, # column
"transferSwitchPoleCount" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.3.1.5",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "2",
"max" : "4"
},
],
"range" : {
"min" : "2",
"max" : "4"
},
},
},
"access" : "readonly",
"description" :
"""The number of poles.""",
}, # column
"transferSwitchAutoReTransferEnabled" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.3.1.20",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readwrite",
"description" :
"""The TS can be configured to automatically retransfer back to the preferred inlet when power is restored.
Re-transfer only occurs if this variable is true.""",
}, # column
"transferSwitchAutoReTransferWaitTime" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.3.1.21",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The Auto Re-Transfer Wait Time. Re-transfer occurs this many seconds after the condition causing the
original transfer has been corrected.""",
}, # column
"transferSwitchAutoReTransferRequiresPhaseSync" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.3.1.22",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readwrite",
"description" :
"""If this parameter is False, then do not retransfer if there is a PHASE_SYNC_FAULT in effect. A
PHASE_SYNC_FAULT occurs when the phase difference between the inlets is too high.""",
}, # column
"transferSwitchFrontPanelManualTransferButtonEnabled" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.3.1.23",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readwrite",
"description" :
"""If this parameter is false, then manual transfers caused by pressing the front panel switch are disabled. """,
}, # column
"transferSwitchCapabilities" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.3.1.24",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Bits",
"overloadStatus" : {
"nodetype" : "namednumber",
"number" : "32"
},
"inletPhaseSyncAngle" : {
"nodetype" : "namednumber",
"number" : "37"
},
"inletPhaseSync" : {
"nodetype" : "namednumber",
"number" : "38"
},
"operatingState" : {
"nodetype" : "namednumber",
"number" : "39"
},
"activeInlet" : {
"nodetype" : "namednumber",
"number" : "40"
},
"switchStatus" : {
"nodetype" : "namednumber",
"number" : "47"
},
},
},
"access" : "readonly",
"description" :
"""A bit string which indicates which sensors are available for the transfer switch.""",
}, # column
"transferSwitchPowerSource1" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.3.1.31",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "RowPointer"},
},
"access" : "readonly",
"description" :
"""This object allows discovery of how the PDU is wired. It indicates the
transferSwitch's first power source which can one of the following:
overCurrentProtector
transfer switch
inlet
If the power source is an OverCurrentProtector, it
contains the OID of an overCurrentProtectorLabel in the
overCurrentProtectorConfiguration Table. The index of the OID can then be
used to reference other objects in the overCurrentProtectorConfigurationTable.
If the power source is an inlet, it contains the OID of
an inletLabel in the inletConfigurationTable. The index of the OID can
then be used to reference other objects in the inletConfigurationTable.
If the power source is a transferSwitch, it
contains the OID of a transferSwitchLabel in the
transferSwitchConfiguration Table. The index of the OID can then be
used to reference other objects in the transferSwitchConfigurationTable. """,
}, # column
"transferSwitchPowerSource2" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.3.1.32",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "RowPointer"},
},
"access" : "readonly",
"description" :
"""This object allows discovery of how the PDU is wired. It indicates the
transferSwitch's second power source which can one of the following:
overCurrentProtector
transfer switch
inlet
If the power source is an OverCurrentProtector, it
contains the OID of an overCurrentProtectorLabel in the
overCurrentProtectorConfiguration Table. The index of the OID can then be
used to reference other objects in the overCurrentProtectorConfigurationTable.
If the power source is an inlet, it contains the OID of
an inletLabel in the inletConfigurationTable. The index of the OID can
then be used to reference other objects in the inletConfigurationTable.
If the power source is a transferSwitch, it
contains the OID of a transferSwitchLabel in the
transferSwitchConfiguration Table. The index of the OID can then be
used to reference other objects in the transferSwitchConfigurationTable """,
}, # column
"transferSwitchSensorConfigurationTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.4",
"status" : "current",
"description" :
"""A list of transfer switch sensor configuration entries. """,
}, # table
"transferSwitchSensorConfigurationEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.4.1",
"status" : "current",
"linkage" : [
"pduId",
"transferSwitchId",
"sensorType",
],
"description" :
"""An entry containing parameters for a transfer switch sensor.""",
}, # row
"transferSwitchSensorLogAvailable" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.4.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readwrite",
"description" :
"""Is logging available for this sensor?""",
}, # column
"transferSwitchSensorUnits" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.4.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorUnitsEnumeration"},
},
"access" : "readonly",
"description" :
"""The base units.
This parameter does not apply to sensors without numerical reading,
e.g. operational state or switch fault sensors.""",
}, # column
"transferSwitchSensorDecimalDigits" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.4.1.7",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The number of digits displayed to the right of the decimal point
This parameter does not apply to sensors without numerical reading,
e.g. operational state or switch fault sensors.""",
}, # column
"transferSwitchSensorAccuracy" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.4.1.8",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "HundredthsOfAPercentage"},
},
"access" : "readonly",
"description" :
"""The accuracy: how close (in percent) the measurement is to the actual value
This parameter does not apply to sensors without numerical reading,
e.g. operational state or switch fault sensors.""",
}, # column
"transferSwitchSensorResolution" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.4.1.9",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The resolution: the minimum difference between any two measured values
The value of this OID variable should be scaled by
transferSwitchSensorDecimalDigits. For example, if the value is 1 and
transferSwitchSensorDecimalDigits is 2, then actual value is 0.01.
This parameter does not apply to sensors without numerical reading,
e.g. operational state or switch fault sensors.""",
}, # column
"transferSwitchSensorTolerance" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.4.1.10",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The resolution: the difference between a sensor value and the actual value
The value of this OID variable should be scaled by
(transferSwitchSensorDecimalDigits + 1). For example, if the value is 50 and
transferSwitchSensorDecimalDigits is 2, then actual value is 0.05.
This parameter does not apply to sensors without numerical reading,
e.g. operational state or switch fault sensors.""",
}, # column
"transferSwitchSensorMaximum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.4.1.11",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The largest possible reading as an unsigned integer.
The value of this OID variable should be scaled by
transferSwitchSensorDecimalDigits. For example, if the value is 1 and
transferSwitchSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled maximum exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading,
e.g. operational state or switch fault sensors.""",
}, # column
"transferSwitchSensorMinimum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.4.1.12",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The smallest possible reading as an unsigned integer.
The value of this OID variable should be scaled by
transferSwitchSensorDecimalDigits. For example, if the value is 1 and
transferSwitchSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading,
e.g. operational state or switch fault sensors.""",
}, # column
"transferSwitchSensorHysteresis" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.4.1.13",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The hysteresis used for deassertions
The value of this OID variable should be scaled by
transferSwitchSensorDecimalDigits. For example, if the value is 1 and
transferSwitchSensorDecimalDigits is 2, then actual value is 0.01.
This parameter does not apply to sensors without numerical reading,
e.g. operational state or switch fault sensors.""",
}, # column
"transferSwitchSensorStateChangeDelay" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.4.1.14",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The delay measured in samples before a state is asserted.
If the value is zero, then the state is asserted as soon as
it is detected; if it is non-zero, say n, then the assertion
condition must exist for n+1 consecutive samples before the corresponding
assertion event is reported.
This parameter does not apply to sensors without numerical reading,
e.g. operational state or switch fault sensors.""",
}, # column
"transferSwitchSensorLowerCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.4.1.21",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The lower critical threshold as an unsigned integer.
The value of this OID variable should be scaled by
transferSwitchSensorDecimalDigits. For example, if the value is 1 and
transferSwitchSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading,
e.g. operational state or switch fault sensors.""",
}, # column
"transferSwitchSensorLowerWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.4.1.22",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The lower warning threshold as an unsigned integer.
The value of this OID variable should be scaled by
transferSwitchSensorDecimalDigits. For example, if the value is 1 and
transferSwitchSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading,
e.g. operational state or switch fault sensors.""",
}, # column
"transferSwitchSensorUpperCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.4.1.23",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The upper critical threshold as an unsigned integer.
The value of this OID variable should be scaled by
transferSwitchSensorDecimalDigits. For example, if the value is 1 and
transferSwitchSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading,
e.g. operational state or switch fault sensors.""",
}, # column
"transferSwitchSensorUpperWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.4.1.24",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The upper warning threshold as an unsigned integer.
The value of this OID variable should be scaled by
transferSwitchSensorDecimalDigits. For example, if the value is 1 and
transferSwitchSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading,
e.g. operational state or switch fault sensors.""",
}, # column
"transferSwitchSensorEnabledThresholds" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.4.1.25",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Bits",
"lowerCritical" : {
"nodetype" : "namednumber",
"number" : "0"
},
"lowerWarning" : {
"nodetype" : "namednumber",
"number" : "1"
},
"upperWarning" : {
"nodetype" : "namednumber",
"number" : "2"
},
"upperCritical" : {
"nodetype" : "namednumber",
"number" : "3"
},
},
},
"access" : "readwrite",
"description" :
"""A bit string which indicates which thresholds are enabled.
This parameter does not apply to sensors without numerical reading,
e.g. operational state or switch fault sensors.""",
}, # column
"transferSwitchSensorSignedMaximum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.4.1.26",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The largest possible reading as a signed integer.
The value of this OID variable should be scaled by
transferSwitchSensorDecimalDigits. For example, if the value is 1 and
transferSwitchSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading,
e.g. operational state or switch fault sensors.""",
}, # column
"transferSwitchSensorSignedMinimum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.4.1.27",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The smallest possible reading as a signed integer.
The value of this OID variable should be scaled by
transferSwitchSensorDecimalDigits. For example, if the value is 1 and
transferSwitchSensorDecimalDigits is 2, then actual value is 0.01.
This value determines whether to use the signed or unsigned threshold
and value columns: If the minimum is below zero the sensor can have
negative readings and the signed columns should be used.
This parameter does not apply to sensors without numerical reading,
e.g. operational state or switch fault sensors.""",
}, # column
"transferSwitchSensorSignedLowerCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.4.1.28",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The lower critical threshold as a signed integer.
The value of this OID variable should be scaled by
transferSwitchSensorDecimalDigits. For example, if the value is 1 and
transferSwitchSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading,
e.g. operational state or switch fault sensors.""",
}, # column
"transferSwitchSensorSignedLowerWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.4.1.29",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The lower warning threshold as a signed integer.
The value of this OID variable should be scaled by
transferSwitchSensorDecimalDigits. For example, if the value is 1 and
transferSwitchSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading,
e.g. operational state or switch fault sensors.""",
}, # column
"transferSwitchSensorSignedUpperCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.4.1.30",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The upper critical threshold as a signed integer.
The value of this OID variable should be scaled by
transferSwitchSensorDecimalDigits. For example, if the value is 1 and
transferSwitchSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading,
e.g. operational state or switch fault sensors.""",
}, # column
"transferSwitchSensorSignedUpperWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.4.1.31",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The upper warning threshold as a signed integer.
The value of this OID variable should be scaled by
transferSwitchSensorDecimalDigits. For example, if the value is 1 and
transferSwitchSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading,
e.g. operational state or switch fault sensors.""",
}, # column
"transferSwitchPoleConfigurationTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.5",
"status" : "current",
"description" :
"""A list of transferSwitch pole configuration entries. The number of
entries is given by the value of transferSwitchPoleCount.""",
}, # table
"transferSwitchPoleConfigurationEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.5.1",
"status" : "current",
"linkage" : [
"pduId",
"transferSwitchId",
"transferSwitchPoleIndex",
],
"description" :
"""An entry containing parametersfor a particular outlet pole.""",
}, # row
"transferSwitchPoleIndex" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.5.1.1",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "256"
},
],
"range" : {
"min" : "1",
"max" : "256"
},
},
},
"access" : "noaccess",
"description" :
"""A unique value for each transferSwitch Pole. Its value
ranges between 1 and the value of transferSwitchPoleCount.""",
}, # column
"transferSwitchPoleLine" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.5.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "LineEnumeration"},
},
"access" : "readonly",
"description" :
"""The Phase for this transferSwitch Pole.""",
}, # column
"transferSwitchPoleIn1Node" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.5.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The node to which this transferSwitch pole Input 1 is connected""",
}, # column
"transferSwitchPoleIn2Node" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.5.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The node to which this transferSwitch pole Input 2 is connected""",
}, # column
"transferSwitchPoleOutNode" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.9.5.1.5",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The node to which this transferSwitch pole Output is connected""",
}, # column
"powerMeter" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.10",
}, # node
"powerMeterConfigurationTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.10.2",
"status" : "current",
"description" :
"""A list of power meter and panel configuration entries.""",
}, # table
"powerMeterConfigurationEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.10.2.1",
"status" : "current",
"linkage" : [
"pduId",
],
"description" :
"""An entry containing configuration objects for power meters and panels.""",
}, # row
"powerMeterPhaseCTRating" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.10.2.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The rating of the Phase CT in A, or 0 if the CT is disabled.
At present, read-only access;
see MIN-ACCESS in MODULE-COMPLIANCE ModulecomplianceRev2""",
}, # column
"powerMeterNeutralCTRating" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.10.2.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The rating of the Neutral CT in A, or 0 if the CT is disabled.
At present, read-only access;
see MIN-ACCESS in MODULE-COMPLIANCE ModulecomplianceRev2""",
}, # column
"powerMeterEarthCTRating" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.10.2.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The rating of the Earth CT in A, or 0 if the CT is disabled.
At present, read-only access;
see MIN-ACCESS in MODULE-COMPLIANCE ModulecomplianceRev2""",
}, # column
"powerMeterBranchCount" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.10.2.1.5",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
""" The maximum number of circuits supported by the panel.
(The number of user configured circuits is in unitConfigurationTable(pduId).circuitCount.)
It can be used to distinguish between power meters and panels.
If value > 0, then panel (BCM)
else power meter""",
}, # column
"powerMeterPanelPositions" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.10.2.1.6",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "256"
},
],
"range" : {
"min" : "1",
"max" : "256"
},
},
},
"access" : "readonly",
"description" :
"""The number of circuit positions in the panel.
Its value is specified by the user when configuring a panel""",
}, # column
"powerMeterPanelLayout" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.10.2.1.7",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "PanelLayoutEnumeration"},
},
"access" : "readonly",
"description" :
""" valid only for panels (powerMeterCircuitPositionCount > 0);
invalid for power meters (powerMeterCircuitPositionCount = 0): value returned is invalid(-1).
The panel circuit position layout:
single column, two columns.""",
}, # column
"powerMeterPanelNumbering" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.10.2.1.8",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "PanelNumberingEnumeration"},
},
"access" : "readonly",
"description" :
""" valid only for panels (powerMeterCircuitPositionCount > 0);
invalid for power meters (powerMeterCircuitPositionCount = 0): value returned is invalid(-1).
The panel circuit position numbering scheme:
odd/even, sequential.""",
}, # column
"powerMeterType" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.10.2.1.9",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "PowerMeterTypeEnumeration"},
},
"access" : "readonly",
"description" :
"""The power meter type:
3-phase, single-phase, split-phase.""",
}, # column
"circuit" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11",
}, # node
"circuitConfigurationTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.2",
"status" : "current",
"description" :
"""A list of circuit configuration entries. The number of
entries is given by the value of panelCircuitPositionCount.""",
}, # table
"circuitConfigurationEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.2.1",
"status" : "current",
"linkage" : [
"pduId",
"circuitId",
],
"description" :
"""An entry containing configuration objects for the circuit.""",
}, # row
"circuitId" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.2.1.1",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "1024"
},
],
"range" : {
"min" : "1",
"max" : "1024"
},
},
},
"access" : "noaccess",
"description" :
"""A unique value for each circuit. circuitId is defined as follows.
It is the circuit position in the panel""",
}, # column
"circuitPoleCount" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.2.1.2",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "256"
},
],
"range" : {
"min" : "1",
"max" : "256"
},
},
},
"access" : "readonly",
"description" :
"""The number of circuit positions (poles) in the circuit.""",
}, # column
"circuitName" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.2.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readwrite",
"description" :
"""The user-defined name for the circuit.
At present, read-only access;
see MIN-ACCESS in MODULE-COMPLIANCE ModulecomplianceRev2""",
}, # column
"circuitType" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.2.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "CircuitTypeEnumeration"},
},
"access" : "readonly",
"description" :
"""The type of circuit:
singlePhaseL_L, singlePhaseL_N, twoPhase,threePhase""",
}, # column
"circuitRatedCurrent" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.2.1.5",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The rating of the breaker for the circuit in A.
At present, read-only access;
see MIN-ACCESS in MODULE-COMPLIANCE ModulecomplianceRev2""",
}, # column
"circuitCTRating" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.2.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The rating of the CTs metering this circuit in A.
At present, read-only access;
see MIN-ACCESS in MODULE-COMPLIANCE ModulecomplianceRev2""",
}, # column
"circuitCapabilities" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.2.1.7",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Bits",
"rmsCurrent" : {
"nodetype" : "namednumber",
"number" : "0"
},
"peakCurrent" : {
"nodetype" : "namednumber",
"number" : "1"
},
"unbalancedCurrent" : {
"nodetype" : "namednumber",
"number" : "2"
},
"rmsVoltage" : {
"nodetype" : "namednumber",
"number" : "3"
},
"activePower" : {
"nodetype" : "namednumber",
"number" : "4"
},
"apparentPower" : {
"nodetype" : "namednumber",
"number" : "5"
},
"powerFactor" : {
"nodetype" : "namednumber",
"number" : "6"
},
"activeEnergy" : {
"nodetype" : "namednumber",
"number" : "7"
},
"apparentEnergy" : {
"nodetype" : "namednumber",
"number" : "8"
},
"surgeProtectorStatus" : {
"nodetype" : "namednumber",
"number" : "21"
},
"frequency" : {
"nodetype" : "namednumber",
"number" : "22"
},
"phaseAngle" : {
"nodetype" : "namednumber",
"number" : "23"
},
"residualCurrent" : {
"nodetype" : "namednumber",
"number" : "25"
},
"rcmState" : {
"nodetype" : "namednumber",
"number" : "26"
},
"reactivePower" : {
"nodetype" : "namednumber",
"number" : "28"
},
"powerQuality" : {
"nodetype" : "namednumber",
"number" : "31"
},
"displacementPowerFactor" : {
"nodetype" : "namednumber",
"number" : "34"
},
},
},
"access" : "readonly",
"description" :
"""A bit string which indicates which sensors are available for the circuit.""",
}, # column
"circuitPoleCapabilities" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.2.1.8",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Bits",
"rmsCurrent" : {
"nodetype" : "namednumber",
"number" : "0"
},
"peakCurrent" : {
"nodetype" : "namednumber",
"number" : "1"
},
"rmsVoltage" : {
"nodetype" : "namednumber",
"number" : "3"
},
"activePower" : {
"nodetype" : "namednumber",
"number" : "4"
},
"apparentPower" : {
"nodetype" : "namednumber",
"number" : "5"
},
"powerFactor" : {
"nodetype" : "namednumber",
"number" : "6"
},
"activeEnergy" : {
"nodetype" : "namednumber",
"number" : "7"
},
"apparentEnergy" : {
"nodetype" : "namednumber",
"number" : "8"
},
"phaseAngle" : {
"nodetype" : "namednumber",
"number" : "23"
},
"rmsVoltageLN" : {
"nodetype" : "namednumber",
"number" : "24"
},
"reactivePower" : {
"nodetype" : "namednumber",
"number" : "28"
},
"displacementPowerFactor" : {
"nodetype" : "namednumber",
"number" : "34"
},
},
},
"access" : "readonly",
"description" :
"""A bit string which indicates which sensors are available for the circuit pole.""",
}, # column
"circuitPowerSource" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.2.1.9",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "RowPointer"},
},
"access" : "readonly",
"description" :
"""This object allows discovery of how the circuit is wired. It indicates the
circuit's power source which can one of the following:
Inlet
It contains the OID of an inletLabel in the inletConfigurationTable.
The index of the OID can
then be used to reference other objects in the inletConfigurationTable.""",
}, # column
"circuitPoleConfigurationTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.3",
"status" : "current",
"description" :
"""A list of Panel Circuit Pole configuration entries. The number of
entries is given by the value of circuitPoleCount for the circuit.""",
}, # table
"circuitPoleConfigurationEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.3.1",
"status" : "current",
"linkage" : [
"pduId",
"circuitId",
"circuitPoleId",
],
"description" :
"""An entry containing configuration objects for the circuit poles.""",
}, # row
"circuitPoleId" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.3.1.1",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "256"
},
],
"range" : {
"min" : "1",
"max" : "256"
},
},
},
"access" : "noaccess",
"description" :
"""A unique value for each circuit Pole. Its value
ranges between 1 and the value of circuitPoleCount.""",
}, # column
"circuitPolePanelPosition" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.3.1.2",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "256"
},
],
"range" : {
"min" : "1",
"max" : "256"
},
},
},
"access" : "readonly",
"description" :
"""The circuit position for this pole.""",
}, # column
"circuitPoleCTNumber" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.3.1.3",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "0",
"max" : "256"
},
],
"range" : {
"min" : "0",
"max" : "256"
},
},
},
"access" : "readwrite",
"description" :
"""The CT Number for this circuit Pole, or 0 if no CT is present.
At present, read-only access;
see MIN-ACCESS in MODULE-COMPLIANCE ModulecomplianceRev2""",
}, # column
"circuitPolePhase" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.3.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "PhaseEnumeration"},
},
"access" : "readwrite",
"description" :
"""The Phase for this circuit Pole.
At present, read-only access;
see MIN-ACCESS in MODULE-COMPLIANCE ModulecomplianceRev2""",
}, # column
"circuitSensorConfigurationTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.4",
"status" : "current",
"description" :
"""A list of configuration entries for an inlet sensor.""",
}, # table
"circuitSensorConfigurationEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.4.1",
"status" : "current",
"linkage" : [
"pduId",
"circuitId",
"sensorType",
],
"description" :
"""An entry containing objects for configuring an inlet sensor.""",
}, # row
"circuitSensorLogAvailable" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.4.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readwrite",
"description" :
"""Is logging enabled for the sensor?""",
}, # column
"circuitSensorUnits" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.4.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorUnitsEnumeration"},
},
"access" : "readonly",
"description" :
"""The base units.
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"circuitSensorDecimalDigits" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.4.1.7",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The number of digits displayed to the right of the decimal point.
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"circuitSensorResolution" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.4.1.9",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The resolution: the minimum difference between any two measured values
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"circuitSensorMaximum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.4.1.11",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The largest possible reading as an unsigned integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled maximum exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"circuitSensorMinimum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.4.1.12",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The smallest possible reading as an unsigned integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"circuitSensorHysteresis" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.4.1.13",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The hysteresis used for deassertions
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"circuitSensorStateChangeDelay" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.4.1.14",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The delay measured in samples before a state is asserted.
If the value is zero, then the state is asserted as soon as
it is detected; if it is non-zero, say n, then the assertion
condition must exist for n+1 consecutive samples before the corresponding
assertion event is reported.
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"circuitSensorLowerCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.4.1.21",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The lower critical threshold as an unsigned integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"circuitSensorLowerWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.4.1.22",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The lower warning threshold as an unsigned integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"circuitSensorUpperCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.4.1.23",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The upper critical threshold as an unsigned integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"circuitSensorUpperWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.4.1.24",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The upper warning threshold as an unsigned integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"circuitSensorEnabledThresholds" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.4.1.25",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Bits",
"lowerCritical" : {
"nodetype" : "namednumber",
"number" : "0"
},
"lowerWarning" : {
"nodetype" : "namednumber",
"number" : "1"
},
"upperWarning" : {
"nodetype" : "namednumber",
"number" : "2"
},
"upperCritical" : {
"nodetype" : "namednumber",
"number" : "3"
},
},
},
"access" : "readwrite",
"description" :
"""A bit string which indicates which thresholds are enabled.
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"circuitSensorSignedMaximum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.4.1.26",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The largest possible reading as a signed integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"circuitSensorSignedMinimum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.4.1.27",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The smallest possible reading as a signed integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
This value determines whether to use the signed or unsigned threshold
and value columns: If the minimum is below zero the sensor can have
negative readings and the signed columns should be used.
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"circuitSensorSignedLowerCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.4.1.28",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The lower critical threshold as a signed integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"circuitSensorSignedLowerWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.4.1.29",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The lower warning threshold as a signed integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"circuitSensorSignedUpperCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.4.1.30",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The upper critical threshold as a signed integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"circuitSensorSignedUpperWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.4.1.31",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The upper warning threshold as a signed integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"circuitPoleSensorConfigurationTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.6",
"status" : "current",
"description" :
"""A list of configuration entries for a circuit's pole sensors.""",
}, # table
"circuitPoleSensorConfigurationEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.6.1",
"status" : "current",
"linkage" : [
"pduId",
"circuitId",
"circuitPoleId",
"sensorType",
],
"description" :
"""An entry containing objects
for configuring an inlet pole sensor.""",
}, # row
"circuitPoleSensorLogAvailable" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.6.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readwrite",
"description" :
"""Is logging enabled for the sensor?""",
}, # column
"circuitPoleSensorUnits" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.6.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorUnitsEnumeration"},
},
"access" : "readonly",
"description" :
"""The base units.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"circuitPoleSensorDecimalDigits" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.6.1.7",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The number of digits displayed to the right of the decimal point.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"circuitPoleSensorResolution" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.6.1.9",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The resolution: the minimum difference between any two measured values
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"circuitPoleSensorMaximum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.6.1.11",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The largest possible reading as an unsigned integer.
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled maximum exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"circuitPoleSensorMinimum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.6.1.12",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The smallest possible reading as an unsigned integer.
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"circuitPoleSensorHysteresis" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.6.1.13",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The hysteresis used for deassertions
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"circuitPoleSensorStateChangeDelay" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.6.1.14",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The delay measured in samples before a state is asserted.
If the value is zero, then the state is asserted as soon as
it is detected; if it is non-zero, say n, then the assertion
condition must exist for n+1 consecutive samples before the corresponding
assertion event is reported.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"circuitPoleSensorLowerCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.6.1.21",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The lower critical threshold as an unsigned integer.
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"circuitPoleSensorLowerWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.6.1.22",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The lower warning threshold as an unsigned integer.
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"circuitPoleSensorUpperCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.6.1.23",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The upper critical threshold as an unsigned integer.
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"circuitPoleSensorUpperWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.6.1.24",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readwrite",
"description" :
"""The upper warning threshold as an unsigned integer.
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
The value is clamped if the scaled threshold exceeds 4294967295. It
is undefined for sensors which can have negative readings.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"circuitPoleSensorEnabledThresholds" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.6.1.25",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Bits",
"lowerCritical" : {
"nodetype" : "namednumber",
"number" : "0"
},
"lowerWarning" : {
"nodetype" : "namednumber",
"number" : "1"
},
"upperWarning" : {
"nodetype" : "namednumber",
"number" : "2"
},
"upperCritical" : {
"nodetype" : "namednumber",
"number" : "3"
},
},
},
"access" : "readwrite",
"description" :
"""A bit string which indicates which thresholds are enabled.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"circuitPoleSensorSignedMaximum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.6.1.26",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The largest possible reading as a signed integer.
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading.""",
}, # column
"circuitPoleSensorSignedMinimum" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.6.1.27",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The smallest possible reading as a signed integer.
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
This value determines whether to use the signed or unsigned threshold
and value columns: If the minimum is below zero the sensor can have
negative readings and the signed columns should be used.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"circuitPoleSensorSignedLowerCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.6.1.28",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The lower critical threshold as a signed integer.
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading.""",
}, # column
"circuitPoleSensorSignedLowerWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.6.1.29",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The lower warning threshold as a signed integer.
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading.""",
}, # column
"circuitPoleSensorSignedUpperCriticalThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.6.1.30",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The upper critical threshold as a signed integer.
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading.""",
}, # column
"circuitPoleSensorSignedUpperWarningThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.3.11.6.1.31",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""The upper warning threshold as a signed integer.
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
The value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading.""",
}, # column
"control" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4",
}, # node
"outletControl" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.1",
}, # node
"outletSwitchControlTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.1.2",
"status" : "current",
"description" :
"""A list of outlets for a PDU. The number of
entries is given by the value of outletCount.""",
}, # table
"outletSwitchControlEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.1.2.1",
"status" : "current",
"linkage" : [
"pduId",
"outletId",
],
"description" :
"""An entry for implementing switching operations on an outlet.""",
}, # row
"switchingOperation" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.1.2.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "OutletSwitchingOperationsEnumeration"},
},
"access" : "readwrite",
"description" :
"""The switching operation.""",
}, # column
"outletSwitchingState" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.1.2.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorStateEnumeration"},
},
"access" : "readonly",
"description" :
"""The outlet state at present""",
}, # column
"outletSwitchingTimeStamp" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.1.2.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The timestamp indicating when the outlet was last switched""",
}, # column
"externalSensorControl" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.2",
}, # node
"transferSwitchControl" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.3",
}, # node
"transferSwitchControlTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.3.1",
"status" : "current",
"description" :
"""A list of transfer switches for a PDU. The number of
entries is given by the value of transferSwitchCount.""",
}, # table
"transferSwitchControlEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.3.1.1",
"status" : "current",
"linkage" : [
"pduId",
"transferSwitchId",
],
"description" :
"""An entry for implementing switching operations on a transfer switch.""",
}, # row
"transferSwitchActiveInlet" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.3.1.1.1",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "64"
},
],
"range" : {
"min" : "1",
"max" : "64"
},
},
},
"access" : "readonly",
"description" :
"""The index of the currently active inlet.""",
}, # column
"transferSwitchTransferToInlet" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.3.1.1.2",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "64"
},
],
"range" : {
"min" : "1",
"max" : "64"
},
},
},
"access" : "readwrite",
"description" :
"""Select the active inlet. If the new inlet is available, it will become
both active and preferred, otherwise an inconsistentValue error will
be returned.
By default the switching operation will fail if the phase difference
between the inlets is too large. In this case the switch can be forced
by writing transferSwitchAlarmOverride as True in the same request.
This variable will always read as 0.""",
}, # column
"transferSwitchAlarmOverride" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.3.1.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readwrite",
"description" :
"""Force transfer even if the phase difference between the inlets is too
large.
This may only be written together with transferSwitchTransferToInlet,
otherwise an inconsistentValue error will be returned. Always reads as
false.""",
}, # column
"transferSwitchLastTransferReason" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.3.1.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "TransferSwitchTransferReasonEnumeration"},
},
"access" : "readonly",
"description" :
"""The reason for the most recent transfer""",
}, # column
"actuatorControl" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.4",
}, # node
"actuatorControlTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.4.2",
"status" : "current",
"description" :
"""A list of actuators for a PDU. """,
}, # table
"actuatorControlEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.4.2.1",
"status" : "current",
"linkage" : [
"pduId",
"sensorID",
],
"description" :
"""An entry for implementing user-initiated state changes for an actuator.""",
}, # row
"actuatorState" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.4.2.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorStateEnumeration"},
},
"access" : "readwrite",
"description" :
"""The actuator state.
A Get operation retrieves the state of the actuator.
A Set operation changes the stae of the sensor.
The valid states for set operations are on and Off.
Attempting to set the state to any other value will generate an error. """,
}, # column
"rcmControl" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.5",
}, # node
"rcmSelfTestTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.5.2",
"status" : "current",
"description" :
"""A list of RCMs. """,
}, # table
"rcmSelfTestEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.5.2.1",
"status" : "current",
"linkage" : [
"pduId",
"inletId",
],
"description" :
"""An entry for implementing self test of an RCM.""",
}, # row
"rcmState" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.5.2.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorStateEnumeration"},
},
"access" : "readwrite",
"description" :
"""The rcm state.
A Get operation retrieves the state of the RCM State Sensor.
A Set operation changes the state of the sensor.
The valid state for set operations is selfTest.
When rcmState is set to selfTest, self test of the RCM starts.
Attempting to set the state to any other value will generate an error.
If the current state is selfTest, then an attempt to set the value to selfTest
will be ignored.
""",
}, # column
"inletSensorControl" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.6",
}, # node
"inletSensorControlTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.6.1",
"status" : "current",
"description" :
"""A list of control entries for the inlet sensors of a PDU. The set
of valid indexes is defined by the value of inletCount and the
inletDeviceCapabilities entry for the selected inlet.""",
}, # table
"inletSensorControlEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.6.1.1",
"status" : "current",
"linkage" : [
"pduId",
"inletId",
"sensorType",
],
"description" :
"""An entry used for controlling an inlet sensor.""",
}, # row
"inletSensorResetValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.6.1.1.1",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""Writing to this variable sets a new value for the addressed sensor. Only
accumulating sensors (e.g. energy counters) can be reset, and currently
the only supported value is 0. Writing any other value returns an error.
This variable will always read as 0.""",
}, # column
"outletSensorControl" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.7",
}, # node
"outletSensorControlTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.7.1",
"status" : "current",
"description" :
"""A list of control entries for the outlet sensors of a PDU. The set
of valid indexes is defined by the value of outletCount and the
outletDeviceCapabilities entry for the selected outlet.""",
}, # table
"outletSensorControlEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.7.1.1",
"status" : "current",
"linkage" : [
"pduId",
"outletId",
"sensorType",
],
"description" :
"""An entry used for controlling an outlet sensor.""",
}, # row
"outletSensorResetValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.7.1.1.1",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""Writing to this variable sets a new value for the addressed sensor. Only
accumulating sensors (e.g. energy counters) can be reset, and currently
the only supported value is 0. Writing any other value returns an error.
This variable will always read as 0.""",
}, # column
"unitSensorControl" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.8",
}, # node
"unitSensorControlTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.8.1",
"status" : "current",
"description" :
"""A list of control entries for the global sensors of a PDU. The set
of valid indexes is defined by the value of pduCount and the
pduDeviceCapabilities entry.""",
}, # table
"unitSensorControlEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.8.1.1",
"status" : "current",
"linkage" : [
"pduId",
"sensorType",
],
"description" :
"""An entry used for controlling an global sensor.""",
}, # row
"unitSensorResetValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.8.1.1.1",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""Writing to this variable sets a new value for the addressed sensor. Only
accumulating sensors (e.g. energy counters) can be reset, and currently
the only supported value is 0. Writing any other value returns an error.
This variable will always read as 0.
This object applies only to multi-inlet PDUs.""",
}, # column
"circuitSensorControl" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.9",
}, # node
"circuitSensorControlTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.9.1",
"status" : "current",
"description" :
"""A list of control entries for the circuit sensors of a BCM2. The maximum
number of entries is given by the value of panelCircuitPositionCount.""",
}, # table
"circuitSensorControlEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.9.1.1",
"status" : "current",
"linkage" : [
"pduId",
"circuitId",
"sensorType",
],
"description" :
"""An entry used for controlling an circuit sensor.""",
}, # row
"circuitSensorResetValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.4.9.1.1.1",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readwrite",
"description" :
"""Writing to this variable sets a new value for the addressed sensor. Only
accumulating sensors (e.g. energy counters) can be reset, and currently
the only supported value is 0. Writing any other value returns an error.
This variable will always read as 0.""",
}, # column
"measurements" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5",
}, # node
"measurementsUnit" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.1",
}, # node
"unitSensorMeasurementsTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.1.3",
"status" : "current",
"description" :
"""A list of unit sensor entries.""",
}, # table
"unitSensorMeasurementsEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.1.3.1",
"status" : "current",
"linkage" : [
"pduId",
"sensorType",
],
"description" :
"""An entry containing measurement objects for an unit sensor.""",
}, # row
"measurementsUnitSensorIsAvailable" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.1.3.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readonly",
"description" :
"""Is the sensor available?""",
}, # column
"measurementsUnitSensorState" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.1.3.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorStateEnumeration"},
},
"access" : "readonly",
"description" :
"""The sensor state.""",
}, # column
"measurementsUnitSensorValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.1.3.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The sensor reading as an unsigned integer.
The value of this OID variable should be scaled by
unitSensorDecimalDigits. For example, if the value is 1 and
unitSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.
This parameter does not apply to sensors without numerical reading,
e.g. power supply status sensors.""",
}, # column
"measurementsUnitSensorTimeStamp" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.1.3.1.5",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The timestamp.""",
}, # column
"measurementsUnitSensorSignedValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.1.3.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The sensor reading as a signed integer.
The value of this OID variable should be scaled by
unitSensorDecimalDigits. For example, if the value is 1 and
unitSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading,
e.g. power supply status sensors.""",
}, # column
"measurementsInlet" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.2",
}, # node
"inletSensorMeasurementsTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.2.3",
"status" : "current",
"description" :
"""A list of inlet sensor entries. The number of
entries is given by the value of inletCount for the PDU.""",
}, # table
"inletSensorMeasurementsEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.2.3.1",
"status" : "current",
"linkage" : [
"pduId",
"inletId",
"sensorType",
],
"description" :
"""An entry containing measurement objects for an inlet sensor.""",
}, # row
"measurementsInletSensorIsAvailable" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.2.3.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readonly",
"description" :
"""Is the sensor available?""",
}, # column
"measurementsInletSensorState" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.2.3.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorStateEnumeration"},
},
"access" : "readonly",
"description" :
"""The sensor state.""",
}, # column
"measurementsInletSensorValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.2.3.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The sensor reading as an unsigned integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"measurementsInletSensorTimeStamp" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.2.3.1.5",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The timestamp.""",
}, # column
"measurementsInletSensorSignedValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.2.3.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The sensor reading as a signed integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"inletPoleSensorMeasurementsTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.2.4",
"status" : "current",
"description" :
"""A list of inletPole sensor entries. The number of
entries is given by the value of inletPoleCount for the inlet.""",
}, # table
"inletPoleSensorMeasurementsEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.2.4.1",
"status" : "current",
"linkage" : [
"pduId",
"inletId",
"inletPoleIndex",
"sensorType",
],
"description" :
"""An entry containing measurement objects for an inletPole sensor.""",
}, # row
"measurementsInletPoleSensorIsAvailable" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.2.4.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readonly",
"description" :
"""Is the sensor available?""",
}, # column
"measurementsInletPoleSensorState" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.2.4.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorStateEnumeration"},
},
"access" : "readonly",
"description" :
"""The sensor state.""",
}, # column
"measurementsInletPoleSensorValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.2.4.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The sensor reading as an unsigned integer.
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"measurementsInletPoleSensorTimeStamp" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.2.4.1.5",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The timestamp.""",
}, # column
"measurementsInletPoleSensorSignedValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.2.4.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The sensor reading as a signed integer.
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading.""",
}, # column
"measurementsOverCurrentProtector" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.3",
}, # node
"overCurrentProtectorSensorMeasurementsTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.3.3",
"status" : "current",
"description" :
"""A list of overCurrentProtector sensor entries. The number of
entries is given by the value of overCurrentProtectorCount for the PDU.""",
}, # table
"overCurrentProtectorSensorMeasurementsEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.3.3.1",
"status" : "current",
"linkage" : [
"pduId",
"overCurrentProtectorIndex",
"sensorType",
],
"description" :
"""An entry containing measurement objects for an overCurrentProtector.""",
}, # row
"measurementsOverCurrentProtectorSensorIsAvailable" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.3.3.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readonly",
"description" :
"""Is the sensor available?""",
}, # column
"measurementsOverCurrentProtectorSensorState" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.3.3.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorStateEnumeration"},
},
"access" : "readonly",
"description" :
"""The sensor state.""",
}, # column
"measurementsOverCurrentProtectorSensorValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.3.3.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The sensor reading as an unsigned integer.
The value of this OID variable should be scaled by
overCurrentProtectorSensorDecimalDigits. For example, if the value is 1 and
overCurrentProtectorSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.
This parameter does not apply to sensors without numerical reading,
e.g. trip sensors.""",
}, # column
"measurementsOverCurrentProtectorSensorTimeStamp" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.3.3.1.5",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The timestamp.""",
}, # column
"measurementsOverCurrentProtectorSensorSignedValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.3.3.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The sensor reading as a signed integer.
The value of this OID variable should be scaled by
overCurrentProtectorSensorDecimalDigits. For example, if the value is 1 and
overCurrentProtectorSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading,
e.g. trip sensors.""",
}, # column
"measurementsOutlet" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.4",
}, # node
"outletSensorMeasurementsTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.4.3",
"status" : "current",
"description" :
"""A list of outlet sensor entries. The number of
entries is given by the value of outletCount for the PDU.""",
}, # table
"outletSensorMeasurementsEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.4.3.1",
"status" : "current",
"linkage" : [
"pduId",
"outletId",
"sensorType",
],
"description" :
"""An entry containing measurement objects for an outlet sensor.""",
}, # row
"measurementsOutletSensorIsAvailable" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.4.3.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readonly",
"description" :
"""Is the sensor available?""",
}, # column
"measurementsOutletSensorState" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.4.3.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorStateEnumeration"},
},
"access" : "readonly",
"description" :
"""The sensor state.""",
}, # column
"measurementsOutletSensorValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.4.3.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The sensor reading as an unsigned integer.
The value of this OID variable should be scaled by
outletSensorDecimalDigits. For example, if the value is 1 and
outletSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.
This parameter does not apply to sensors without numerical reading,
e.g. outlet state sensors.""",
}, # column
"measurementsOutletSensorTimeStamp" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.4.3.1.5",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The timestamp.""",
}, # column
"measurementsOutletSensorSignedValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.4.3.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The sensor reading as a signed integer.
The value of this OID variable should be scaled by
outletSensorDecimalDigits. For example, if the value is 1 and
outletSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading,
e.g. outlet state sensors.""",
}, # column
"outletPoleSensorMeasurementsTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.4.4",
"status" : "current",
"description" :
"""A list of outletPole sensor entries. The number of
entries is given by the value of outletPoletCount for the outlet.""",
}, # table
"outletPoleSensorMeasurementsEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.4.4.1",
"status" : "current",
"linkage" : [
"pduId",
"outletId",
"outletPoleIndex",
"sensorType",
],
"description" :
"""An entry containing measurement objects for an outletPole sensor.""",
}, # row
"measurementsOutletPoleSensorIsAvailable" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.4.4.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readonly",
"description" :
"""Is the sensor available?""",
}, # column
"measurementsOutletPoleSensorState" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.4.4.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorStateEnumeration"},
},
"access" : "readonly",
"description" :
"""The sensor state.""",
}, # column
"measurementsOutletPoleSensorValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.4.4.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The sensor reading as an unsigned integer.
The value of this OID variable should be scaled by
outletPoleSensorDecimalDigits. For example, if the value is 50 and
outletPoleSensorDecimalDigits is 2, then actual value is 0.05.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.
This parameter does not apply to sensors without numerical reading.""",
}, # column
"measurementsOutletPoleSensorTimeStamp" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.4.4.1.5",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The timestamp.""",
}, # column
"measurementsOutletPoleSensorSignedValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.4.4.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The sensor reading as a signed integer.
The value of this OID variable should be scaled by
outletPoleSensorDecimalDigits. For example, if the value is 50 and
outletPoleSensorDecimalDigits is 2, then actual value is 0.05.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading.""",
}, # column
"measurementsExternalSensor" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.5",
}, # node
"externalSensorMeasurementsTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.5.3",
"status" : "current",
"description" :
"""A list of external sensor entries. The number of
entries is given by the value of externalSensorCount for the PDU.""",
}, # table
"externalSensorMeasurementsEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.5.3.1",
"status" : "current",
"linkage" : [
"pduId",
"sensorID",
],
"description" :
"""An entry containing measurement objects for an external sensor.""",
}, # row
"measurementsExternalSensorIsAvailable" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.5.3.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readonly",
"description" :
"""Is the sensor available?""",
}, # column
"measurementsExternalSensorState" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.5.3.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorStateEnumeration"},
},
"access" : "readonly",
"description" :
"""The sensor state.""",
}, # column
"measurementsExternalSensorValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.5.3.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The sensor reading as a signed integer.
The value of this OID variable should be scaled by
externalSensorDecimalDigits. For example, if the value is 1 and
externalSensorDecimalDigits is 2, then actual value is 0.01.
This parameter does not apply to sensors without numerical reading,
e.g. contact closure or smoke detection sensors.""",
}, # column
"measurementsExternalSensorTimeStamp" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.5.3.1.5",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The sensor reading timestamp.""",
}, # column
"measurementsWire" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.6",
}, # node
"wireSensorMeasurementsTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.6.3",
"status" : "deprecated",
"description" :
"""A list of wire sensor entries. The number of
entries is given by the value of wireCount for the PDU.""",
}, # table
"wireSensorMeasurementsEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.6.3.1",
"status" : "deprecated",
"linkage" : [
"pduId",
"wireId",
"sensorType",
],
"description" :
"""An entry containing measurement objects for a wire.""",
}, # row
"measurementsWireSensorIsAvailable" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.6.3.1.2",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readonly",
"description" :
"""Is the sensor available?""",
}, # column
"measurementsWireSensorState" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.6.3.1.3",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorStateEnumeration"},
},
"access" : "readonly",
"description" :
"""The sensor state.""",
}, # column
"measurementsWireSensorValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.6.3.1.4",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The sensor value.
The value of this OID variable should be scaled by
wireSensorDecimalDigits. For example, if the value is 1 and
wireSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the value exceeds 4294967295
This parameter does not apply to sensors without numerical reading.""",
}, # column
"measurementsWireSensorTimeStamp" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.6.3.1.5",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The timestamp.""",
}, # column
"measurementsTransferSwitch" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.7",
}, # node
"transferSwitchSensorMeasurementsTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.7.3",
"status" : "current",
"description" :
"""A list of transfer switch sensor entries. The number of
entries is given by the value of transferSwitchCount for the PDU.""",
}, # table
"transferSwitchSensorMeasurementsEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.7.3.1",
"status" : "current",
"linkage" : [
"pduId",
"transferSwitchId",
"sensorType",
],
"description" :
"""An entry containing measurement objects for a transfer switch.""",
}, # row
"measurementsTransferSwitchSensorIsAvailable" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.7.3.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readonly",
"description" :
"""Is the sensor available?""",
}, # column
"measurementsTransferSwitchSensorState" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.7.3.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorStateEnumeration"},
},
"access" : "readonly",
"description" :
"""The sensor state.""",
}, # column
"measurementsTransferSwitchSensorValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.7.3.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The sensor reading as an unsigned integer.
The value of this OID variable should be scaled by
transferSwitchSensorDecimalDigits. For example, if the value is 1 and
transferSwitchSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.
This parameter does not apply to sensors without numerical reading,
e.g. operational state or switch fault sensors.""",
}, # column
"measurementsTransferSwitchSensorTimeStamp" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.7.3.1.5",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The timestamp.""",
}, # column
"measurementsTransferSwitchSensorSignedValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.7.3.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The sensor reading as a signed integer.
The value of this OID variable should be scaled by
transferSwitchSensorDecimalDigits. For example, if the value is 1 and
transferSwitchSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading,
e.g. operational state or switch fault sensors.""",
}, # column
"measurementsCircuit" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.8",
}, # node
"circuitSensorMeasurementsTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.8.3",
"status" : "current",
"description" :
"""A list of circuit sensor entries. """,
}, # table
"circuitSensorMeasurementsEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.8.3.1",
"status" : "current",
"linkage" : [
"pduId",
"circuitId",
"sensorType",
],
"description" :
"""An entry containing measurement objects for circuit sensor.""",
}, # row
"measurementsCircuitSensorIsAvailable" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.8.3.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readonly",
"description" :
"""Is the sensor available?""",
}, # column
"measurementsCircuitSensorState" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.8.3.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorStateEnumeration"},
},
"access" : "readonly",
"description" :
"""The sensor state.""",
}, # column
"measurementsCircuitSensorValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.8.3.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The sensor reading as an unsigned integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"measurementsCircuitSensorTimeStamp" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.8.3.1.5",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The timestamp.""",
}, # column
"measurementsCircuitSensorSignedValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.8.3.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The sensor reading as a signed integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"circuitPoleSensorMeasurementsTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.8.4",
"status" : "current",
"description" :
"""A list of panel circuit pole sensor entries. The number of
entries is given by the value of inletCount for the PDU.""",
}, # table
"circuitPoleSensorMeasurementsEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.8.4.1",
"status" : "current",
"linkage" : [
"pduId",
"circuitId",
"circuitPoleId",
"sensorType",
],
"description" :
"""An entry containing measurement objects for a circuit pole sensor.""",
}, # row
"measurementsCircuitPoleSensorIsAvailable" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.8.4.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readonly",
"description" :
"""Is the sensor available?""",
}, # column
"measurementsCircuitPoleSensorState" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.8.4.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorStateEnumeration"},
},
"access" : "readonly",
"description" :
"""The sensor state.""",
}, # column
"measurementsCircuitPoleSensorValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.8.4.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The sensor reading as an unsigned integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"measurementsCircuitPoleSensorTimeStamp" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.8.4.1.5",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The timestamp.""",
}, # column
"measurementsCircuitPoleSensorSignedValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.5.8.4.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The sensor reading as a signed integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).
This parameter does not apply to sensors without numerical reading,
e.g. surge protector status sensors.""",
}, # column
"log" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6",
}, # node
"logUnit" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.1",
}, # node
"logIndexTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.1.1",
"status" : "current",
"description" :
"""A table of log entries.""",
}, # table
"logIndexEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.1.1.1",
"status" : "current",
"linkage" : [
"pduId",
],
"description" :
"""An entry in the log containing sensor data.""",
}, # row
"oldestLogID" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.1.1.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The index of the oldest data in the buffer for this PDU.""",
}, # column
"newestLogID" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.1.1.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The index of the newest data in the buffer for this PDU.""",
}, # column
"logTimeStampTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.1.2",
"status" : "current",
"description" :
"""A list of entries containing the timestamps of the entries in the log.""",
}, # table
"logTimeStampEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.1.2.1",
"status" : "current",
"linkage" : [
"pduId",
"logIndex",
],
"description" :
"""An entry containing the timestamp for log entries.""",
}, # row
"logIndex" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.1.2.1.1",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "256"
},
],
"range" : {
"min" : "1",
"max" : "256"
},
},
},
"access" : "noaccess",
"description" :
"""A unique value for each entry in the log. Its value
ranges between 1 and the value of logSize.""",
}, # column
"logTimeStamp" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.1.2.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The time when the data was collected. It is measured in seconds relative to
January 1, 1970 (midnight UTC/GMT), i.e a value of 0 indicates
January 1, 1970 (midnight UTC/GMT).""",
}, # column
"unitSensorLogTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.1.3",
"status" : "current",
"description" :
"""A list of unit sensor entries in the log.""",
}, # table
"unitSensorLogEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.1.3.1",
"status" : "current",
"linkage" : [
"pduId",
"sensorType",
"logIndex",
],
"description" :
"""An entry containing log objects for an unit sensor.""",
}, # row
"logUnitSensorDataAvailable" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.1.3.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readonly",
"description" :
"""Is data available for this sensor during this measurement period?""",
}, # column
"logUnitSensorState" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.1.3.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorStateEnumeration"},
},
"access" : "readonly",
"description" :
"""The sensor state.""",
}, # column
"logUnitSensorAvgValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.1.3.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The average sensor reading for the log period as an unsigned integer.
The value of this OID variable should be scaled by
unitSensorDecimalDigits. For example, if the value is 1 and
unitSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.""",
}, # column
"logUnitSensorMaxValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.1.3.1.5",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The maximum sensor reading for the log period as an unsigned integer.
The value of this OID variable should be scaled by
unitSensorDecimalDigits. For example, if the value is 1 and
unitSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.""",
}, # column
"logUnitSensorMinValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.1.3.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The minimum sensor reading for the log period as an unsigned integer.
The value of this OID variable should be scaled by
unitSensorDecimalDigits. For example, if the value is 1 and
unitSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.""",
}, # column
"logUnitSensorSignedAvgValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.1.3.1.7",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The average sensor reading for the log period as a signed integer.
The value of this OID variable should be scaled by
unitSensorDecimalDigits. For example, if the value is 1 and
unitSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).""",
}, # column
"logUnitSensorSignedMaxValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.1.3.1.8",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The maximum sensor reading for the log period as a signed integer.
The value of this OID variable should be scaled by
unitSensorDecimalDigits. For example, if the value is 1 and
unitSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).""",
}, # column
"logUnitSensorSignedMinValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.1.3.1.9",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The minimum sensor reading for the log period as a signed integer.
The value of this OID variable should be scaled by
unitSensorDecimalDigits. For example, if the value is 1 and
unitSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).""",
}, # column
"logInlet" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.2",
}, # node
"inletSensorLogTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.2.3",
"status" : "current",
"description" :
"""A list of inlet sensor entries. The number of
entries is given by the value of inletCount for the PDU.""",
}, # table
"inletSensorLogEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.2.3.1",
"status" : "current",
"linkage" : [
"pduId",
"inletId",
"sensorType",
"logIndex",
],
"description" :
"""An entry containing log objects for an inlet sensor.""",
}, # row
"logInletSensorDataAvailable" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.2.3.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readonly",
"description" :
"""Is data available for this sensor during this measurement period?""",
}, # column
"logInletSensorState" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.2.3.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorStateEnumeration"},
},
"access" : "readonly",
"description" :
"""The sensor state.""",
}, # column
"logInletSensorAvgValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.2.3.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The average sensor reading for the log period as an unsigned integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.""",
}, # column
"logInletSensorMaxValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.2.3.1.5",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The maximum sensor reading for the log period as an unsigned integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.""",
}, # column
"logInletSensorMinValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.2.3.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The minimum sensor reading for the log period as an unsigned integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.""",
}, # column
"logInletSensorSignedAvgValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.2.3.1.7",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The average sensor reading for the log period as a signed integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).""",
}, # column
"logInletSensorSignedMaxValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.2.3.1.8",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The maximum sensor reading for the log period as a signed integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).""",
}, # column
"logInletSensorSignedMinValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.2.3.1.9",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The minimum sensor reading for the log period as a signed integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).""",
}, # column
"inletPoleSensorLogTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.2.4",
"status" : "current",
"description" :
"""A list of inletPole sensor entries. The number of
entries is given by the value of inletPoleCount for the inlet.""",
}, # table
"inletPoleSensorLogEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.2.4.1",
"status" : "current",
"linkage" : [
"pduId",
"inletId",
"inletPoleIndex",
"sensorType",
"logIndex",
],
"description" :
"""An entry containing log objects for an inletPole sensor.""",
}, # row
"logInletPoleSensorDataAvailable" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.2.4.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readonly",
"description" :
"""Is data available for this sensor during this measurement period?""",
}, # column
"logInletPoleSensorState" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.2.4.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorStateEnumeration"},
},
"access" : "readonly",
"description" :
"""The sensor state.""",
}, # column
"logInletPoleSensorAvgValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.2.4.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The average sensor reading for the log period as an unsigned integer.
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.""",
}, # column
"logInletPoleSensorMaxValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.2.4.1.5",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The maximum sensor reading for the log period as an unsigned integer.
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.""",
}, # column
"logInletPoleSensorMinValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.2.4.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The minimum sensor reading for the log period as an unsigned integer.
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.""",
}, # column
"logInletPoleSensorSignedAvgValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.2.4.1.7",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The average sensor reading for the log period as a signed integer.
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).""",
}, # column
"logInletPoleSensorSignedMaxValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.2.4.1.8",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The maximum sensor reading for the log period as a signed integer.
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).""",
}, # column
"logInletPoleSensorSignedMinValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.2.4.1.9",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The minimum sensor reading for the log period as a signed integer.
The value of this OID variable should be scaled by
inletPoleSensorDecimalDigits. For example, if the value is 1 and
inletPoleSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).""",
}, # column
"logOverCurrentProtector" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.3",
}, # node
"overCurrentProtectorSensorLogTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.3.3",
"status" : "current",
"description" :
"""A list of overCurrentProtector sensor entries. The number of
entries is given by the value of overCurrentProtectorCount for the PDU.""",
}, # table
"overCurrentProtectorSensorLogEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.3.3.1",
"status" : "current",
"linkage" : [
"pduId",
"overCurrentProtectorIndex",
"sensorType",
"logIndex",
],
"description" :
"""An entry containing log objects for an overCurrentProtector sensor.""",
}, # row
"logOverCurrentProtectorSensorDataAvailable" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.3.3.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readonly",
"description" :
"""Is data available for this sensor during this measurement period?""",
}, # column
"logOverCurrentProtectorSensorState" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.3.3.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorStateEnumeration"},
},
"access" : "readonly",
"description" :
"""The sensor state.""",
}, # column
"logOverCurrentProtectorSensorAvgValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.3.3.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The average sensor reading for the log period as an unsigned integer.
The value of this OID variable should be scaled by
overCurrentProtectorSensorDecimalDigits. For example, if the value is 1 and
overCurrentProtectorSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.""",
}, # column
"logOverCurrentProtectorSensorMaxValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.3.3.1.5",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The maximum sensor reading for the log period as an unsigned integer.
The value of this OID variable should be scaled by
overCurrentProtectorSensorDecimalDigits. For example, if the value is 1 and
overCurrentProtectorSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.""",
}, # column
"logOverCurrentProtectorSensorMinValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.3.3.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The minimum sensor reading for the log period as an unsigned integer.
The value of this OID variable should be scaled by
overCurrentProtectorSensorDecimalDigits. For example, if the value is 1 and
overCurrentProtectorSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.""",
}, # column
"logOverCurrentProtectorSensorSignedAvgValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.3.3.1.7",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The average sensor reading for the log period as a signed integer.
The value of this OID variable should be scaled by
overCurrentProtectorSensorDecimalDigits. For example, if the value is 1 and
overCurrentProtectorSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).""",
}, # column
"logOverCurrentProtectorSensorSignedMaxValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.3.3.1.8",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The maximum sensor reading for the log period as a signed integer.
The value of this OID variable should be scaled by
overCurrentProtectorSensorDecimalDigits. For example, if the value is 1 and
overCurrentProtectorSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).""",
}, # column
"logOverCurrentProtectorSensorSignedMinValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.3.3.1.9",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The minimum sensor reading for the log period as a signed integer.
The value of this OID variable should be scaled by
overCurrentProtectorSensorDecimalDigits. For example, if the value is 1 and
overCurrentProtectorSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).""",
}, # column
"logOutlet" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.4",
}, # node
"outletSensorLogTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.4.3",
"status" : "current",
"description" :
"""A list of outlet sensor entries. The number of
entries is given by the value of outletCount for the PDU.""",
}, # table
"outletSensorLogEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.4.3.1",
"status" : "current",
"linkage" : [
"pduId",
"outletId",
"sensorType",
"logIndex",
],
"description" :
"""An entry containing log objects for an outlet sensor.""",
}, # row
"logOutletSensorDataAvailable" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.4.3.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readonly",
"description" :
"""Is data available for this sensor during this measurement period?""",
}, # column
"logOutletSensorState" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.4.3.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorStateEnumeration"},
},
"access" : "readonly",
"description" :
"""The sensor state.""",
}, # column
"logOutletSensorAvgValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.4.3.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The average sensor reading for the log period as an unsigned integer.
The value of this OID variable should be scaled by
outletSensorDecimalDigits. For example, if the value is 1 and
outletSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.""",
}, # column
"logOutletSensorMaxValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.4.3.1.5",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The maximum sensor reading for the log period as an unsigned integer.
The value of this OID variable should be scaled by
outletSensorDecimalDigits. For example, if the value is 1 and
outletSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.""",
}, # column
"logOutletSensorMinValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.4.3.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The minimum sensor reading for the log period as an unsigned integer.
The value of this OID variable should be scaled by
outletSensorDecimalDigits. For example, if the value is 1 and
outletSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.""",
}, # column
"logOutletSensorSignedAvgValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.4.3.1.7",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The average sensor reading for the log period as a signed integer.
The value of this OID variable should be scaled by
outletSensorDecimalDigits. For example, if the value is 1 and
outletSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).""",
}, # column
"logOutletSensorSignedMaxValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.4.3.1.8",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The maximum sensor reading for the log period as a signed integer.
The value of this OID variable should be scaled by
outletSensorDecimalDigits. For example, if the value is 1 and
outletSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).""",
}, # column
"logOutletSensorSignedMinValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.4.3.1.9",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The minimum sensor reading for the log period as a signed integer.
The value of this OID variable should be scaled by
outletSensorDecimalDigits. For example, if the value is 1 and
outletSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).""",
}, # column
"outletPoleSensorLogTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.4.4",
"status" : "current",
"description" :
"""A list of outletPole sensor entries. The number of
entries is given by the value of outletPoleCount for the outlet.""",
}, # table
"outletPoleSensorLogEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.4.4.1",
"status" : "current",
"linkage" : [
"pduId",
"outletId",
"outletPoleIndex",
"sensorType",
"logIndex",
],
"description" :
"""An entry containing log objects for an outletPole sensor.""",
}, # row
"logOutletPoleSensorDataAvailable" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.4.4.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readonly",
"description" :
"""Is data available for this sensor during this measurement period?""",
}, # column
"logOutletPoleSensorState" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.4.4.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorStateEnumeration"},
},
"access" : "readonly",
"description" :
"""The sensor state.""",
}, # column
"logOutletPoleSensorAvgValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.4.4.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The average sensor reading for the log period as an unsigned integer.
The value of this OID variable should be scaled by
outletPoleSensorDecimalDigits. For example, if the value is 1 and
outletPoleSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.""",
}, # column
"logOutletPoleSensorMaxValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.4.4.1.5",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The maximum sensor reading for the log period as an unsigned integer.
The value of this OID variable should be scaled by
outletPoleSensorDecimalDigits. For example, if the value is 1 and
outletPoleSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.""",
}, # column
"logOutletPoleSensorMinValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.4.4.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The minimum sensor reading for the log period as an unsigned integer.
The value of this OID variable should be scaled by
outletPoleSensorDecimalDigits. For example, if the value is 1 and
outletPoleSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.""",
}, # column
"logOutletPoleSensorSignedAvgValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.4.4.1.7",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The average sensor reading for the log period as a signed integer.
The value of this OID variable should be scaled by
outletPoleSensorDecimalDigits. For example, if the value is 1 and
outletPoleSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).""",
}, # column
"logOutletPoleSensorSignedMaxValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.4.4.1.8",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The maximum sensor reading for the log period as a signed integer.
The value of this OID variable should be scaled by
outletPoleSensorDecimalDigits. For example, if the value is 1 and
outletPoleSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).""",
}, # column
"logOutletPoleSensorSignedMinValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.4.4.1.9",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The minimum sensor reading for the log period as a signed integer.
The value of this OID variable should be scaled by
outletPoleSensorDecimalDigits. For example, if the value is 1 and
outletPoleSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).""",
}, # column
"logExternalSensor" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.5",
}, # node
"externalSensorLogTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.5.3",
"status" : "current",
"description" :
"""A list of external sensor entries. The number of
entries is given by the value of externalSensorCount for the PDU.""",
}, # table
"externalSensorLogEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.5.3.1",
"status" : "current",
"linkage" : [
"pduId",
"sensorID",
"logIndex",
],
"description" :
"""An entry containing log objects for an external sensor.""",
}, # row
"logExternalSensorDataAvailable" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.5.3.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readonly",
"description" :
"""Is data available for this sensor during this measurement period?""",
}, # column
"logExternalSensorState" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.5.3.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorStateEnumeration"},
},
"access" : "readonly",
"description" :
"""The sensor state.""",
}, # column
"logExternalSensorAvgValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.5.3.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The sensor reading average value.
The value of this OID variable should be scaled by
externalSensorDecimalDigits. For example, if the value is 1 and
externalSensorDecimalDigits is 2, then actual value is 0.01.""",
}, # column
"logExternalSensorMaxValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.5.3.1.5",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The sensor reading maximum value.
The value of this OID variable should be scaled by
externalSensorDecimalDigits. For example, if the value is 1 and
externalSensorDecimalDigits is 2, then actual value is 0.01.""",
}, # column
"logExternalSensorMinValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.5.3.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The sensor reading minimum value.
The value of this OID variable should be scaled by
externalSensorDecimalDigits. For example, if the value is 1 and
externalSensorDecimalDigits is 2, then actual value is 0.01.""",
}, # column
"logWire" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.6",
}, # node
"wireSensorLogTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.6.3",
"status" : "deprecated",
"description" :
"""A list of wire sensor entries. The number of
entries is given by the value of wireCount for the PDU.""",
}, # table
"wireSensorLogEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.6.3.1",
"status" : "deprecated",
"linkage" : [
"pduId",
"wireId",
"sensorType",
"logIndex",
],
"description" :
"""An entry containing log objects for a wire sensor.""",
}, # row
"logWireSensorDataAvailable" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.6.3.1.2",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readonly",
"description" :
"""Is data available for this sensor during this measurement period?""",
}, # column
"logWireSensorState" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.6.3.1.3",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorStateEnumeration"},
},
"access" : "readonly",
"description" :
"""The sensor state.""",
}, # column
"logWireSensorAvgValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.6.3.1.4",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The sensor reading average value.
The value of this OID variable should be scaled by
wireSensorDecimalDigits. For example, if the value is 1 and
wireSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the value exceeds 4294967295""",
}, # column
"logWireSensorMaxValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.6.3.1.5",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The sensor reading maximum value.
The value of this OID variable should be scaled by
wireSensorDecimalDigits. For example, if the value is 1 and
wireSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the value exceeds 4294967295""",
}, # column
"logWireSensorMinValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.6.3.1.6",
"status" : "deprecated",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The sensor reading minimum value.
The value of this OID variable should be scaled by
wireSensorDecimalDigits. For example, if the value is 1 and
wireSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the value exceeds 4294967295.""",
}, # column
"logTransferSwitch" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.7",
}, # node
"transferSwitchSensorLogTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.7.3",
"status" : "current",
"description" :
"""A list of Transfer Switch sensor entries. The number of
entries is given by the value of transferSwitchCount for the PDU.""",
}, # table
"transferSwitchSensorLogEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.7.3.1",
"status" : "current",
"linkage" : [
"pduId",
"transferSwitchId",
"sensorType",
"logIndex",
],
"description" :
"""An entry containing log objects for a transfer switch sensor.""",
}, # row
"logTransferSwitchSensorDataAvailable" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.7.3.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readonly",
"description" :
"""Is data available for this sensor during this measurement period?""",
}, # column
"logTransferSwitchSensorState" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.7.3.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorStateEnumeration"},
},
"access" : "readonly",
"description" :
"""The sensor state.""",
}, # column
"logTransferSwitchSensorAvgValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.7.3.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The average sensor reading for the log period as an unsigned integer.
The value of this OID variable should be scaled by
transferSwitchSensorDecimalDigits. For example, if the value is 1 and
transferSwitchSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.""",
}, # column
"logTransferSwitchSensorMaxValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.7.3.1.5",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The maximum sensor reading for the log period as an unsigned integer.
The value of this OID variable should be scaled by
transferSwitchSensorDecimalDigits. For example, if the value is 1 and
transferSwitchSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.""",
}, # column
"logTransferSwitchSensorMinValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.7.3.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The minimum sensor reading for the log period as an unsigned integer.
The value of this OID variable should be scaled by
transferSwitchSensorDecimalDigits. For example, if the value is 1 and
transferSwitchSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.""",
}, # column
"logTransferSwitchSensorSignedAvgValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.7.3.1.7",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The average sensor reading for the log period as a signed integer.
The value of this OID variable should be scaled by
transferSwitchSensorDecimalDigits. For example, if the value is 1 and
transferSwitchSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).""",
}, # column
"logTransferSwitchSensorSignedMaxValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.7.3.1.8",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The maximum sensor reading for the log period as a signed integer.
The value of this OID variable should be scaled by
transferSwitchSensorDecimalDigits. For example, if the value is 1 and
transferSwitchSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).""",
}, # column
"logTransferSwitchSensorSignedMinValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.7.3.1.9",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The minimum sensor reading for the log period as a signed integer.
The value of this OID variable should be scaled by
transferSwitchSensorDecimalDigits. For example, if the value is 1 and
transferSwitchSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).""",
}, # column
"logCircuit" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.8",
}, # node
"circuitSensorLogTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.8.3",
"status" : "current",
"description" :
"""A list of Circuit sensor log entries. The number of
entries is given by the value of panelinletCount for the Floor PDU.""",
}, # table
"circuitSensorLogEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.8.3.1",
"status" : "current",
"linkage" : [
"pduId",
"circuitId",
"sensorType",
"logIndex",
],
"description" :
"""An entry containing log objects for a circuit sensor.""",
}, # row
"logCircuitSensorDataAvailable" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.8.3.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readonly",
"description" :
"""Is data available for this sensor during this measurement period?""",
}, # column
"logCircuitSensorState" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.8.3.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorStateEnumeration"},
},
"access" : "readonly",
"description" :
"""The sensor state.""",
}, # column
"logCircuitSensorAvgValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.8.3.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The average sensor reading for the log period as an unsigned integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.""",
}, # column
"logCircuitSensorMaxValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.8.3.1.5",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The maximum sensor reading for the log period as an unsigned integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.""",
}, # column
"logCircuitSensorMinValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.8.3.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The minimum sensor reading for the log period as an unsigned integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.""",
}, # column
"logCircuitSensorSignedAvgValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.8.3.1.7",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The average sensor reading for the log period as a signed integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).""",
}, # column
"logCircuitSensorSignedMaxValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.8.3.1.8",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The maximum sensor reading for the log period as a signed integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).""",
}, # column
"logCircuitSensorSignedMinValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.8.3.1.9",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The minimum sensor reading for the log period as a signed integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).""",
}, # column
"circuitPoleSensorLogTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.8.5",
"status" : "current",
"description" :
"""A list of circuit pole sensor log entries. The number of
entries is given by the value of panelCircuitPoleCount for the circut.""",
}, # table
"circuitPoleSensorLogEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.8.5.1",
"status" : "current",
"linkage" : [
"pduId",
"circuitId",
"circuitPoleId",
"sensorType",
"logIndex",
],
"description" :
"""An entry containing log objects for a circuit pole sensor.""",
}, # row
"logCircuitPoleSensorDataAvailable" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.8.5.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "TruthValue"},
},
"access" : "readonly",
"description" :
"""Is data available for this sensor during this measurement period?""",
}, # column
"logCircuitPoleSensorState" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.8.5.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"PDU2-MIB", "name" : "SensorStateEnumeration"},
},
"access" : "readonly",
"description" :
"""The sensor state.""",
}, # column
"logCircuitPoleSensorAvgValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.8.5.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The average sensor reading for the log period as an unsigned integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.""",
}, # column
"logCircuitPoleSensorMaxValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.8.5.1.5",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The maximum sensor reading for the log period as an unsigned integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.""",
}, # column
"logCircuitPoleSensorMinValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.8.5.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The minimum sensor reading for the log period as an unsigned integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
This value will wrap around if the scaled sensor reading exceeds
4294967295. It is undefined for sensors which can have negative
readings.""",
}, # column
"logCircuitPoleSensorSignedAvgValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.8.5.1.7",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The average sensor reading for the log period as a signed integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).""",
}, # column
"logCircuitPoleSensorSignedMaxValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.8.5.1.8",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The maximum sensor reading for the log period as a signed integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).""",
}, # column
"logCircuitPoleSensorSignedMinValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.6.8.5.1.9",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Integer32"},
},
"access" : "readonly",
"description" :
"""The minimum sensor reading for the log period as a signed integer.
The value of this OID variable should be scaled by
inletSensorDecimalDigits. For example, if the value is 1 and
inletSensorDecimalDigits is 2, then actual value is 0.01.
This value is undefined for sensors whose range exceeds the
Integer32 range (-2147483648 .. 2147483647).""",
}, # column
"conformance" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.9",
}, # node
"compliances" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.9.1",
}, # node
"groups" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.9.2",
}, # node
"reliability" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.10",
}, # node
"reliabilityData" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.10.1",
}, # node
"reliabilityDataTableSequenceNumber" : {
"nodetype" : "scalar",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.10.1.1",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "2147483647"
},
],
"range" : {
"min" : "1",
"max" : "2147483647"
},
},
},
"access" : "readonly",
"description" :
"""The sequence number for updates to the reliability data table""",
}, # scalar
"reliabilityDataTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.10.1.2",
"status" : "current",
"description" :
"""A list of PDU reliability data entries.""",
}, # table
"reliabilityDataEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.10.1.2.1",
"status" : "current",
"linkage" : [
"reliabilityIndex",
],
"description" :
"""An entry containing reliability data for a particular PDU.""",
}, # row
"reliabilityIndex" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.10.1.2.1.1",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "4096"
},
],
"range" : {
"min" : "1",
"max" : "4096"
},
},
},
"access" : "noaccess",
"description" :
"""Index of the entry in the table.""",
}, # column
"reliabilityId" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.10.1.2.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""Unique ID of the entry.
POH
Power on hours.
CB.<label>.TRIPCNT
Trip count of circuit breaker with label <label>.
CTRL.<serial>.<addr>.MASTER.CSUMERRLASTHOUR
Number of checksum errors in slave (controller board) to master (CPU
board) communication in the last hour on controller with serial
number <serial> and bus address <addr>.
CTRL.<serial>.<addr>.SLAVE.CSUMERRLASTHOUR
Number of checksum errors in master (CPU board) to slave (controller
board) communication in the last hour on controller with serial
number <serial> and bus address <addr>.
CTRL.<serial>.<addr>.TOUTLASTHOUR
Number of communication timeouts to controller with serial
number <serial> and bus address <addr> in the last hour.
CTRL.<serial>.<addr>.RLY.<num>.CYCLECNT
Number of cycles the relay <num> on the controller board with serial
number <serial> and bus address <addr> has made. A cycle is an
off->on followed by an on->off event later. The count is increased on
the off->on transition.
CTRL.<serial>.<addr>.RLY.<num>.FAILLASTHOUR
Number of failed switching operations on relay <num> on the controller
board with serial number <serial> and bus address <addr> in the last
hour. It depends on the specific controller board hardware and what
error conditions are detected.""",
}, # column
"reliabilityDataValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.10.1.2.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The normalized value""",
}, # column
"reliabilityDataMaxPossible" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.10.1.2.1.4",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The maximum possible normalized value""",
}, # column
"reliabilityDataWorstValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.10.1.2.1.5",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The worst normalized value seen so far""",
}, # column
"reliabilityDataThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.10.1.2.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The normalized Threshold value """,
}, # column
"reliabilityDataRawUpperBytes" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.10.1.2.1.7",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The Upper 4 bytes of the raw ( not normalized) data.
reliabilityDataRawUpperBytes and reliabilityDataRawLowerBytes
should be combined and interpreted as a signed 64-bit value""",
}, # column
"reliabilityDataRawLowerBytes" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.10.1.2.1.8",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The lower 4 bytes of the raw ( not normalized) data.
reliabilityDataRawUpperBytes and reliabilityDataRawLowerBytes
should be combined and interpreted as a signed 64-bit value""",
}, # column
"reliabilityDataFlags" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.10.1.2.1.9",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Bits",
"invalidFlag" : {
"nodetype" : "namednumber",
"number" : "0"
},
"oldValue" : {
"nodetype" : "namednumber",
"number" : "1"
},
"criticalEntry" : {
"nodetype" : "namednumber",
"number" : "2"
},
},
},
"access" : "readonly",
"description" :
"""Flags""",
}, # column
"reliabilityErrorLog" : {
"nodetype" : "node",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.10.2",
}, # node
"reliabilityErrorLogTable" : {
"nodetype" : "table",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.10.2.2",
"status" : "current",
"description" :
"""A list of PDU reliability ErrorLog entries. """,
}, # table
"reliabilityErrorLogEntry" : {
"nodetype" : "row",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.10.2.2.1",
"status" : "current",
"linkage" : [
"reliabilityErrorLogIndex",
],
"description" :
"""An entry containing reliability ErrorLog data for a particular PDU.""",
}, # row
"reliabilityErrorLogIndex" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.10.2.2.1.1",
"status" : "current",
"syntax" : {
"type" : {
"basetype" : "Integer32",
"ranges" : [
{
"min" : "1",
"max" : "2147483647"
},
],
"range" : {
"min" : "1",
"max" : "2147483647"
},
},
},
"access" : "noaccess",
"description" :
"""Index of the entry in the table.""",
}, # column
"reliabilityErrorLogId" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.10.2.2.1.2",
"status" : "current",
"syntax" : {
"type" : { "module" :"SNMPv2-TC", "name" : "DisplayString"},
},
"access" : "readonly",
"description" :
"""Unique ID of the entry.
POH
Power on hours.
CB.<label>.TRIPCNT
Trip count of circuit breaker with label <label>.
CTRL.<serial>.<addr>.MASTER.CSUMERRLASTHOUR
Number of checksum errors in slave (controller board) to master (CPU
board) communication in the last hour on controller with serial
number <serial> and bus address <addr>.
CTRL.<serial>.<addr>.SLAVE.CSUMERRLASTHOUR
Number of checksum errors in master (CPU board) to slave (controller
board) communication in the last hour on controller with serial
number <serial> and bus address <addr>.
CTRL.<serial>.<addr>.TOUTLASTHOUR
Number of communication timeouts to controller with serial
number <serial> and bus address <addr> in the last hour.
CTRL.<serial>.<addr>.RLY.<num>.CYCLECNT
Number of cycles the relay <num> on the controller board with serial
number <serial> and bus address <addr> has made. A cycle is an
off->on followed by an on->off event later. The count is increased on
the off->on transition.
CTRL.<serial>.<addr>.RLY.<num>.FAILLASTHOUR
Number of failed switching operations on relay <num> on the controller
board with serial number <serial> and bus address <addr> in the last
hour. It depends on the specific controller board hardware and what
error conditions are detected.""",
}, # column
"reliabilityErrorLogValue" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.10.2.2.1.3",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The normalized value""",
}, # column
"reliabilityErrorLogThreshold" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.10.2.2.1.6",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The normalized Threshold value """,
}, # column
"reliabilityErrorLogRawUpperBytes" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.10.2.2.1.7",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The Upper 4 bytes of the raw ( not normalized) data.
reliabilityDataRawUpperBytes and reliabilityDataRawLowerBytes
should be combined and interpreted as a signed 64-bit value""",
}, # column
"reliabilityErrorLogRawLowerBytes" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.10.2.2.1.8",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The lower 4 bytes of the raw ( not normalized) data.
reliabilityDataRawUpperBytes and reliabilityDataRawLowerBytes
should be combined and interpreted as a signed 64-bit value""",
}, # column
"reliabilityErrorLogPOH" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.10.2.2.1.9",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The time of occurrence of the event measured from the last
time the PDU was powered on""",
}, # column
"reliabilityErrorLogTime" : {
"nodetype" : "column",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.10.2.2.1.10",
"status" : "current",
"syntax" : {
"type" : { "module" :"", "name" : "Unsigned32"},
},
"access" : "readonly",
"description" :
"""The UTC time of occurrence of the event """,
}, # column
}, # nodes
"notifications" : {
"systemStarted" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.1",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""The system has started. """,
}, # notification
"systemReset" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.2",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""The system was reset. The conditions under which
this trap is sent include, but are not limited to, the following.
using the GUI - Maintenance->Reset Unit
using the CLI - reset pdu unit""",
}, # notification
"userLogin" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.3",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""A user logged in.""",
}, # notification
"userLogout" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.4",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""A user logged out.""",
}, # notification
"userAuthenticationFailure" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.5",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""A user authentication attempt failed.""",
}, # notification
"userSessionTimeout" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.8",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""A user session timed out.""",
}, # notification
"userAdded" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.11",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"targetUser" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""A user was added to the system.""",
}, # notification
"userModified" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.12",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"targetUser" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""A user was modified.""",
}, # notification
"userDeleted" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.13",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"targetUser" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""A user was deleted from the system.""",
}, # notification
"roleAdded" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.14",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"roleName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""A role was added to the system.""",
}, # notification
"roleModified" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.15",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"roleName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""A role was modified.""",
}, # notification
"roleDeleted" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.16",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"roleName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""A role was deleted from the system.""",
}, # notification
"deviceUpdateStarted" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.20",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"imageVersion" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""The device update has started.""",
}, # notification
"deviceUpdateCompleted" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.21",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"imageVersion" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""The device update has completed.""",
}, # notification
"userBlocked" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.22",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""A blocked user tried to log in.""",
}, # notification
"powerControl" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.23",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"outletLabel" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsOutletSensorState" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"switchingOperation" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""An outlet switching operation has been performed.
Note that measurementsOutletSensorState represents the state of the outlet at the time the trap was sent.
This may be different from the final state of the outlet. For instance,
if the outlet is cycled and the outlet cycle delay is 20 seconds, this variable will
indicate OFF although the final state of the outlet will be ON. The final state of the
outlet will be indicated in the outletSensorStateChange trap for the outlet onOff sensor.""",
}, # notification
"userPasswordChanged" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.24",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"targetUser" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""User password was changed.""",
}, # notification
"passwordSettingsChanged" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.28",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""Strong password settings changed.""",
}, # notification
"firmwareValidationFailed" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.38",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""Firmware validation failed.""",
}, # notification
"logFileCleared" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.41",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""The log file has been cleared.""",
}, # notification
"bulkConfigurationSaved" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.53",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""Bulk Configuration saved.""",
}, # notification
"bulkConfigurationCopied" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.54",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""Bulk Configuration copied to the device.""",
}, # notification
"pduSensorStateChange" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.60",
"status" : "deprecated",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pduNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"typeOfSensor" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsUnitSensorTimeStamp" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsUnitSensorValue" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsUnitSensorState" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsUnitSensorSignedValue" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"oldSensorState" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""PDU Sensor State Change.
Which value field to use depends on the metadata of the sensor.
measurementsUnitSensorValue is undefined for sensors which can
have negative readings, measurementsUnitSensorSignedValue is
undefined for sensors whose range exceeds the Integer32 range.
Both fields do not apply to sensors without numerical readings.""",
}, # notification
"inletSensorStateChange" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.61",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pduNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"inletLabel" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"typeOfSensor" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsInletSensorTimeStamp" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsInletSensorValue" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsInletSensorState" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsInletSensorSignedValue" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"oldSensorState" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""Inlet Sensor State Change.
Which value field to use depends on the metadata of the sensor.
measurementsInletSensorValue is undefined for sensors which can
have negative readings, measurementsInletSensorSignedValue is
undefined for sensors whose range exceeds the Integer32 range.
Both fields do not apply to sensors without numerical readings.
For an rcmState sensor transitioning to the fail state, the value is the residual current
just before the sensor transitioned to the fail state i.e.
if typeOfSensor = rcmState and measurementsInletSensorState = fail,
then measurementsInletSensorValue = the residual current just before the sensor transitioned to the fail state""",
}, # notification
"inletPoleSensorStateChange" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.62",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pduNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"inletLabel" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"inletPoleNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"typeOfSensor" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsInletPoleSensorTimeStamp" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsInletPoleSensorValue" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsInletPoleSensorState" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsInletPoleSensorSignedValue" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"oldSensorState" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""Inlet Pole Sensor State Change.
Which value field to use depends on the metadata of the sensor.
measurementsInletPoleSensorValue is undefined for sensors which can
have negative readings, measurementsInletPoleSensorSignedValue is
undefined for sensors whose range exceeds the Integer32 range.
Both fields do not apply to sensors without numerical readings.""",
}, # notification
"outletSensorStateChange" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.63",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pduNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"outletLabel" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"typeOfSensor" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsOutletSensorTimeStamp" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsOutletSensorValue" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsOutletSensorState" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsOutletSensorSignedValue" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"oldSensorState" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""Outlet Sensor State Change.
Which value field to use depends on the metadata of the sensor.
measurementsOutletSensorValue is undefined for sensors which can
have negative readings, measurementsOutletSensorSignedValue is
undefined for sensors whose range exceeds the Integer32 range.
Both fields do not apply to sensors without numerical readings.""",
}, # notification
"outletPoleSensorStateChange" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.64",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pduNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"outletLabel" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"outletPoleNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"typeOfSensor" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsOutletPoleSensorTimeStamp" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsOutletPoleSensorValue" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsOutletPoleSensorState" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsOutletPoleSensorSignedValue" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"oldSensorState" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""Outlet Pole Sensor State Change.
Which value field to use depends on the metadata of the sensor.
measurementsOutletPoleSensorValue is undefined for sensors which can
have negative readings, measurementsOutletPoleSensorSignedValue is
undefined for sensors whose range exceeds the Integer32 range.
Both fields do not apply to sensors without numerical readings.""",
}, # notification
"overCurrentProtectorSensorStateChange" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.65",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pduNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"overCurrentProtectorLabel" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"typeOfSensor" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsOverCurrentProtectorSensorTimeStamp" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsOverCurrentProtectorSensorValue" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsOverCurrentProtectorSensorState" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsOverCurrentProtectorSensorSignedValue" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"oldSensorState" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""Overcurrent Protector Sensor State Change.
Which value field to use depends on the metadata of the sensor.
measurementsOverCurrentProtectorSensorValue is undefined for sensors which can
have negative readings, measurementsOverCurrentProtectorSensorSignedValue is
undefined for sensors whose range exceeds the Integer32 range.
Both fields do not apply to sensors without numerical readings.""",
}, # notification
"externalSensorStateChange" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.66",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pduNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"externalSensorNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"typeOfSensor" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsExternalSensorTimeStamp" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsExternalSensorValue" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsExternalSensorState" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"oldSensorState" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"externalSensorSerialNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"externalOnOffSensorSubtype" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"externalSensorChannelNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""External Sensor State Change.""",
}, # notification
"smtpMessageTransmissionFailure" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.67",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"smtpMessageRecipients" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"smtpServer" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""SMTP message transmission failure""",
}, # notification
"ldapError" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.68",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"errorDescription" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""LDAP Error occurred; errorDescription describes the error""",
}, # notification
"deviceUpdateFailed" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.70",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"imageVersion" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""The device update has failed.""",
}, # notification
"loadSheddingModeEntered" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.71",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""The PX has enetered Load Shedding Mode""",
}, # notification
"loadSheddingModeExited" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.72",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""The PX has exited Load Shedding Mode""",
}, # notification
"pingServerEnabled" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.73",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"serverIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""The ping feature has been enabled""",
}, # notification
"pingServerDisabled" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.74",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"serverIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""The ping feature has been disabled""",
}, # notification
"serverNotReachable" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.75",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"serverIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""The server is not reachable""",
}, # notification
"serverReachable" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.76",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"serverIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""The server is reachable""",
}, # notification
"rfCodeTagConnected" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.77",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""The RF Code Tag is Connected""",
}, # notification
"rfCodeTagDisconnected" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.78",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""The RF Code Tag is Disconnected""",
}, # notification
"deviceIdentificationChanged" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.79",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"deviceChangedParameter" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"changedParameterNewValue" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""Device identification has changed""",
}, # notification
"usbSlaveConnected" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.80",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""USB Connectivity to slave has been established""",
}, # notification
"usbSlaveDisconnected" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.81",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""USB Connectivity to slave has been lost""",
}, # notification
"lhxSupportChanged" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.82",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"lhxSupportEnabled" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
},
"description" :
"""The Schroff LHX Support has been either enabled or disabled.""",
}, # notification
"userAcceptedRestrictedServiceAgreement" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.83",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""The user accepted the Restricted Service Agreement.""",
}, # notification
"userDeclinedRestrictedServiceAgreement" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.84",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""The user declined the Restricted Service Agreement.""",
}, # notification
"wireSensorStateChange" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.85",
"status" : "deprecated",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pduNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"wireLabel" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"typeOfSensor" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsWireSensorTimeStamp" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsWireSensorValue" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsWireSensorState" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"oldSensorState" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""Wire Sensor State Change.""",
}, # notification
"transferSwitchSensorStateChange" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.86",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pduNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"transferSwitchLabel" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"typeOfSensor" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsTransferSwitchSensorTimeStamp" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsTransferSwitchSensorValue" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsTransferSwitchSensorState" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsTransferSwitchSensorSignedValue" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"oldSensorState" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"transferSwitchLastTransferReason" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
},
"description" :
"""Transfer Switch Sensor State Change.
Which value field to use depends on the metadata of the sensor.
measurementsTransferSwitchSensorValue is undefined for sensors which can
have negative readings, measurementsTransferSwitchSensorSignedValue is
undefined for sensors whose range exceeds the Integer32 range.
Both fields do not apply to sensors without numerical readings.""",
}, # notification
"deviceSettingsSaved" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.88",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""Device Settings have been saved.""",
}, # notification
"deviceSettingsRestored" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.89",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""Device Settings have been restored.""",
}, # notification
"webcamInserted" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.90",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"webcamModel" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"webcamConnectionPort" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""A webcam has been inserted""",
}, # notification
"webcamRemoved" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.91",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"webcamModel" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"webcamConnectionPort" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""A webcam has been removed""",
}, # notification
"inletEnabled" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.92",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"inletLabel" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""PDU operation has been enabled for an inlet""",
}, # notification
"inletDisabled" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.93",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"inletLabel" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""PDU operation has been disabled for an inlet""",
}, # notification
"serverConnectivityUnrecoverable" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.94",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"serverIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""The connection to the server could not be recovered""",
}, # notification
"radiusError" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.95",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"errorDescription" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""LDAP Error occurred; errorDescription describes the error""",
}, # notification
"serverReachabilityError" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.96",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"serverIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"errorDescription" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""Server Reachability Error occurred; errorDescription describes the error.
This trap is sent when the Error state is entered.
Reasons for transitioning to the Error state include the following:
Unable to resolve the server hostname. """,
}, # notification
"inletSensorReset" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.97",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pduNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"inletLabel" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"typeOfSensor" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""An inlet sensor was reset.""",
}, # notification
"outletSensorReset" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.98",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pduNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"outletLabel" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"typeOfSensor" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""An outlet sensor was reset.""",
}, # notification
"unknownPeripheralDeviceAttached" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.99",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"peripheralDeviceRomcode" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"peripheralDevicePackagePosition" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""An unknown peripheral device was attached.""",
}, # notification
"peripheralDeviceFirmwareUpdate" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.100",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"peripheralDevicePackageSerialNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"peripheralDeviceFirmwareUpdateState" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"peripheralDevicePackageFirmwareVersion" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""The firmware update state of a peripheral device changed while
performing update to peripheralDevicePackageFirmwareVersion.""",
}, # notification
"unitSensorReset" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.101",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pduNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"typeOfSensor" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""A global sensor was reset.""",
}, # notification
"unitSensorStateChange" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.102",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pduNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"typeOfSensor" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsUnitSensorTimeStamp" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsUnitSensorValue" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsUnitSensorState" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsUnitSensorSignedValue" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"oldSensorState" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""Unit Sensor State Change.
Which value field to use depends on the metadata of the sensor.
measurementsUnitSensorValue is undefined for sensors which can
have negative readings, measurementsUnitSensorSignedValue is
undefined for sensors whose range exceeds the Integer32 range.
Both fields do not apply to sensors without numerical readings.""",
}, # notification
"circuitSensorStateChange" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.103",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pduNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"circuitNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"typeOfSensor" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsCircuitSensorTimeStamp" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsCircuitSensorValue" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsCircuitSensorState" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsCircuitSensorSignedValue" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"oldSensorState" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""circuit Sensor State Change.
Which value field to use depends on the metadata of the sensor.
measurementsOutletSensorValue is undefined for sensors which can
have negative readings, measurementsCircuitSensorSignedValue is
undefined for sensors whose range exceeds the Integer32 range.
Both fields do not apply to sensors without numerical readings.""",
}, # notification
"circuitPoleSensorStateChange" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.104",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pduNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"circuitNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"circuitPoleNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"typeOfSensor" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsCircuitPoleSensorTimeStamp" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsCircuitPoleSensorValue" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsCircuitPoleSensorState" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"measurementsCircuitPoleSensorSignedValue" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"oldSensorState" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""Circuit Pole Sensor State Change.
Which value field to use depends on the metadata of the sensor.
measurementsOutletPoleSensorValue is undefined for sensors which can
have negative readings, measurementsCircuitPoleSensorSignedValue is
undefined for sensors whose range exceeds the Integer32 range.
Both fields do not apply to sensors without numerical readings.""",
}, # notification
"circuitAdded" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.105",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pduNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"circuitNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"circuitName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"circuitType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"circuitRatedCurrent" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"circuitCTRating" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""A Circuit has been added""",
}, # notification
"circuitDeleted" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.106",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pduNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"circuitNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"circuitName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"circuitType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""A Circuit has been deleted""",
}, # notification
"circuitModified" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.107",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pduNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"circuitNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"circuitName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"circuitType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"circuitRatedCurrent" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"circuitCTRating" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""A Circuit has been modified""",
}, # notification
"circuitSensorReset" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.108",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pduNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"circuitNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"typeOfSensor" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""An ciruit sensor was reset.""",
}, # notification
"powerMeterAdded" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.109",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pduNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"powerMeterPhaseCTRating" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"powerMeterNeutralCTRating" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"powerMeterEarthCTRating" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"powerMeterPanelPositions" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"powerMeterPanelLayout" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"powerMeterPanelNumbering" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"powerMeterType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""A Power Meter has been added""",
}, # notification
"powerMeterDeleted" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.110",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pduNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"powerMeterType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""A Power Meter has been deleted""",
}, # notification
"powerMeterModified" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.111",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pduNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"userName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"powerMeterPhaseCTRating" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"powerMeterNeutralCTRating" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"powerMeterEarthCTRating" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"powerMeterType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""A Power Meter has been modified""",
}, # notification
"smsMessageTransmissionFailure" : {
"nodetype" : "notification",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.0.112",
"status" : "current",
"objects" : {
"pduName" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"phoneNumber" : {
"nodetype" : "object",
"module" : "PDU2-MIB"
},
"sysContact" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysName" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
"sysLocation" : {
"nodetype" : "object",
"module" : "RFC1213-MIB"
},
},
"description" :
"""Sending an SMS message failed.""",
}, # notification
}, # notifications
"groups" : {
"configGroup" : {
"nodetype" : "group",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.9.2.1",
"status" : "current",
"members" : {
"pduCount" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"pduManufacturer" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"pduModel" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"pduSerialNumber" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"pduRatedVoltage" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"pduRatedCurrent" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"pduRatedFrequency" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"pduRatedVA" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"pduImage" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletCount" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchCount" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"productType" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"overCurrentProtectorCount" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletCount" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletControllerCount" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletControllerCount" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"meteringControllerCount" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorCount" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitCount" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"pxMACAddress" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"utcOffset" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"pduName" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"boardVersion" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"boardFirmwareVersion" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"boardFirmwareTimeStamp" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"unitSensorLogAvailable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"unitSensorUnits" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"unitSensorDecimalDigits" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"unitSensorResolution" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"unitSensorMaximum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"unitSensorMinimum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"unitSensorHysteresis" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"unitSensorStateChangeDelay" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"unitSensorLowerCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"unitSensorLowerWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"unitSensorUpperCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"unitSensorUpperWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"unitSensorEnabledThresholds" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"unitSensorSignedMaximum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"unitSensorSignedMinimum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"unitSensorSignedLowerCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"unitSensorSignedLowerWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"unitSensorSignedUpperCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"unitSensorSignedUpperWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletLabel" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletName" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletPlug" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletPoleCount" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletRatedVoltage" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletRatedCurrent" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletDeviceCapabilities" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletPoleCapabilities" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletPlugDescriptor" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletEnableState" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletPoleLine" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletPoleNode" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletSensorLogAvailable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletSensorUnits" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletSensorDecimalDigits" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletSensorResolution" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletSensorMaximum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletSensorMinimum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletSensorHysteresis" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletSensorStateChangeDelay" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletSensorLowerCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletSensorLowerWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletSensorUpperCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletSensorUpperWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletSensorEnabledThresholds" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletSensorSignedMaximum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletSensorSignedMinimum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletSensorSignedLowerCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletSensorSignedLowerWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletSensorSignedUpperCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletSensorSignedUpperWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletPoleSensorLogAvailable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletPoleSensorUnits" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletPoleSensorDecimalDigits" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletPoleSensorResolution" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletPoleSensorMaximum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletPoleSensorMinimum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletPoleSensorHysteresis" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletPoleSensorStateChangeDelay" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletPoleSensorLowerCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletPoleSensorLowerWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletPoleSensorUpperCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletPoleSensorUpperWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletPoleSensorEnabledThresholds" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletPoleSensorSignedMaximum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletPoleSensorSignedMinimum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletPoleSensorSignedLowerCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletPoleSensorSignedLowerWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletPoleSensorSignedUpperCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletPoleSensorSignedUpperWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletRCMResidualOperatingCurrent" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"overCurrentProtectorLabel" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"overCurrentProtectorName" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"overCurrentProtectorType" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"overCurrentProtectorRatedCurrent" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"overCurrentProtectorCapabilities" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"overCurrentProtectorPoleCount" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"overCurrentProtectorPoleLine" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"overCurrentProtectorPoleInNode" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"overCurrentProtectorPoleOutNode" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"overCurrentProtectorSensorLogAvailable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"overCurrentProtectorSensorUnits" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"overCurrentProtectorSensorDecimalDigits" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"overCurrentProtectorSensorResolution" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"overCurrentProtectorSensorMaximum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"overCurrentProtectorSensorMinimum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"overCurrentProtectorSensorHysteresis" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"overCurrentProtectorSensorStateChangeDelay" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"overCurrentProtectorSensorLowerCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"overCurrentProtectorSensorLowerWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"overCurrentProtectorSensorUpperCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"overCurrentProtectorSensorUpperWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"overCurrentProtectorSensorEnabledThresholds" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"overCurrentProtectorSensorSignedMaximum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"overCurrentProtectorSensorSignedMinimum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"overCurrentProtectorSensorSignedLowerCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"overCurrentProtectorSensorSignedLowerWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"overCurrentProtectorSensorSignedUpperCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"overCurrentProtectorSensorSignedUpperWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"overCurrentProtectorPowerSource" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletLabel" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletName" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletReceptacle" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletPoleCount" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletRatedVoltage" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletRatedCurrent" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletRatedVA" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletDeviceCapabilities" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletPoleCapabilities" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletPowerCyclingPowerOffPeriod" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletStateOnStartup" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletUseGlobalPowerCyclingPowerOffPeriod" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletSwitchable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletReceptacleDescriptor" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletNonCritical" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletSequenceDelay" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletPoleLine" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletPoleNode" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletSensorLogAvailable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletSensorUnits" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletSensorDecimalDigits" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletSensorResolution" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletSensorMaximum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletSensorMinimum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletSensorHysteresis" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletSensorStateChangeDelay" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletSensorLowerCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletSensorLowerWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletSensorUpperCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletSensorUpperWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletSensorEnabledThresholds" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletSensorSignedMaximum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletSensorSignedMinimum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletSensorSignedLowerCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletSensorSignedLowerWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletSensorSignedUpperCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletSensorSignedUpperWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletPoleSensorLogAvailable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletPoleSensorUnits" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletPoleSensorDecimalDigits" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletPoleSensorResolution" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletPoleSensorMaximum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletPoleSensorMinimum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletPoleSensorHysteresis" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletPoleSensorStateChangeDelay" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletPoleSensorLowerCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletPoleSensorLowerWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletPoleSensorUpperCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletPoleSensorUpperWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletPoleSensorEnabledThresholds" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletPoleSensorSignedMaximum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletPoleSensorSignedMinimum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletPoleSensorSignedLowerCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletPoleSensorSignedLowerWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletPoleSensorSignedUpperCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletPoleSensorSignedUpperWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletPowerSource" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorType" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorSerialNumber" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorName" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorDescription" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorXCoordinate" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorYCoordinate" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorZCoordinate" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorChannelNumber" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalOnOffSensorSubtype" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorLogAvailable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorUnits" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorDecimalDigits" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorResolution" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorMaximum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorMinimum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorHysteresis" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorStateChangeDelay" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorLowerCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorLowerWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorUpperCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorUpperWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorEnabledThresholds" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorsZCoordinateUnits" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorIsActuator" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorPosition" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorUseDefaultThresholds" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorTypeDefaultHysteresis" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorTypeDefaultStateChangeDelay" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorTypeDefaultLowerCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorTypeDefaultLowerWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorTypeDefaultUpperCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorTypeDefaultUpperWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorTypeDefaultEnabledThresholds" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementPeriod" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsPerLogEntry" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logSize" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"unitDeviceCapabilities" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"globalOutletPowerCyclingPowerOffPeriod" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"globalOutletStateOnStartup" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"relayBehaviorOnPowerLoss" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"pduPowerCyclingPowerOffPeriod" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"pduDaisychainMemberType" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"managedExternalSensorCount" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletPowerupSequence" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"loadShedding" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"serverCount" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"serverIPAddress" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"serverPingEnabled" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inrushGuardDelay" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"cascadedDeviceConnected" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"synchronizeWithNTPServer" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"useDHCPProvidedNTPServer" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"firstNTPServerAddressType" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"firstNTPServerAddress" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"secondNTPServerAddressType" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"secondNTPServerAddress" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchLabel" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchName" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchPreferredInlet" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchPoleCount" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchAutoReTransferEnabled" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchAutoReTransferWaitTime" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchAutoReTransferRequiresPhaseSync" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchFrontPanelManualTransferButtonEnabled" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchCapabilities" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchPoleLine" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchPoleIn1Node" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchPoleIn2Node" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchPoleOutNode" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchSensorLogAvailable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchSensorUnits" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchSensorDecimalDigits" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchSensorResolution" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchSensorMaximum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchSensorMinimum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchSensorHysteresis" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchSensorStateChangeDelay" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchSensorLowerCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchSensorLowerWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchSensorUpperCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchSensorUpperWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchSensorEnabledThresholds" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchSensorSignedMaximum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchSensorSignedMinimum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchSensorSignedLowerCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchSensorSignedLowerWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchSensorSignedUpperCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchSensorSignedUpperWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchPowerSource1" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchPowerSource2" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"peripheralDevicePackageSerialNumber" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"peripheralDevicePackageModel" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"peripheralDevicePackageFirmwareVersion" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"peripheralDevicePackageMinFirmwareVersion" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"peripheralDevicePackageFirmwareTimeStamp" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"peripheralDevicePackagePosition" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"peripheralDevicePackageState" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"deviceCascadeType" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"deviceCascadePosition" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"peripheralDevicesAutoManagement" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorAlarmedToNormalDelay" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"frontPanelOutletSwitching" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"frontPanelRCMSelfTest" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"frontPanelActuatorControl" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"powerMeterPanelPositions" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"powerMeterPanelLayout" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"powerMeterPanelNumbering" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"powerMeterPhaseCTRating" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"powerMeterNeutralCTRating" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"powerMeterEarthCTRating" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"powerMeterBranchCount" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"powerMeterType" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitPoleCount" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitName" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitType" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitRatedCurrent" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitCTRating" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitCapabilities" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitPoleCapabilities" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitPowerSource" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitPolePanelPosition" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitPoleCTNumber" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitPolePhase" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitSensorLogAvailable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitSensorUnits" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitSensorDecimalDigits" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitSensorResolution" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitSensorMaximum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitSensorMinimum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitSensorHysteresis" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitSensorStateChangeDelay" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitSensorLowerCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitSensorLowerWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitSensorUpperCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitSensorUpperWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitSensorEnabledThresholds" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitSensorSignedMaximum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitSensorSignedMinimum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitSensorSignedLowerCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitSensorSignedLowerWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitSensorSignedUpperCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitSensorSignedUpperWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitPoleSensorLogAvailable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitPoleSensorUnits" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitPoleSensorDecimalDigits" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitPoleSensorResolution" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitPoleSensorMaximum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitPoleSensorMinimum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitPoleSensorHysteresis" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitPoleSensorStateChangeDelay" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitPoleSensorLowerCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitPoleSensorLowerWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitPoleSensorUpperCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitPoleSensorUpperWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitPoleSensorEnabledThresholds" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitPoleSensorSignedMaximum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitPoleSensorSignedMinimum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitPoleSensorSignedLowerCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitPoleSensorSignedLowerWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitPoleSensorSignedUpperCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitPoleSensorSignedUpperWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"networkInterfaceType" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"activeDNSServerAddressType" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"activeDNSServerAddress" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"activeDNSServerAddressSource" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"activeDNSServerCount" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
}, # members
"description" :
"""A collection of objects representing configuration data.""",
}, # group
"logGroup" : {
"nodetype" : "group",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.9.2.2",
"status" : "current",
"members" : {
"dataLogging" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"oldestLogID" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"newestLogID" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logTimeStamp" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"dataLoggingEnableForAllSensors" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logUnitSensorDataAvailable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logUnitSensorState" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logUnitSensorAvgValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logUnitSensorMaxValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logUnitSensorMinValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logUnitSensorSignedAvgValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logUnitSensorSignedMaxValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logUnitSensorSignedMinValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logInletSensorDataAvailable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logInletSensorState" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logInletSensorAvgValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logInletSensorMaxValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logInletSensorMinValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logInletSensorSignedAvgValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logInletSensorSignedMaxValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logInletSensorSignedMinValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logInletPoleSensorDataAvailable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logInletPoleSensorState" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logInletPoleSensorAvgValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logInletPoleSensorMaxValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logInletPoleSensorMinValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logInletPoleSensorSignedAvgValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logInletPoleSensorSignedMaxValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logInletPoleSensorSignedMinValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logOutletSensorDataAvailable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logOutletSensorState" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logOutletSensorAvgValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logOutletSensorMaxValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logOutletSensorMinValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logOutletSensorSignedAvgValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logOutletSensorSignedMaxValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logOutletSensorSignedMinValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logOutletPoleSensorDataAvailable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logOutletPoleSensorState" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logOutletPoleSensorAvgValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logOutletPoleSensorMaxValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logOutletPoleSensorMinValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logOutletPoleSensorSignedAvgValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logOutletPoleSensorSignedMaxValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logOutletPoleSensorSignedMinValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logOverCurrentProtectorSensorDataAvailable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logOverCurrentProtectorSensorState" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logOverCurrentProtectorSensorAvgValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logOverCurrentProtectorSensorMaxValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logOverCurrentProtectorSensorMinValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logOverCurrentProtectorSensorSignedAvgValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logOverCurrentProtectorSensorSignedMaxValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logOverCurrentProtectorSensorSignedMinValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logExternalSensorDataAvailable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logExternalSensorState" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logExternalSensorAvgValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logExternalSensorMaxValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logExternalSensorMinValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logTransferSwitchSensorDataAvailable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logTransferSwitchSensorState" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logTransferSwitchSensorAvgValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logTransferSwitchSensorMaxValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logTransferSwitchSensorMinValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logTransferSwitchSensorSignedAvgValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logTransferSwitchSensorSignedMaxValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logTransferSwitchSensorSignedMinValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logCircuitSensorDataAvailable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logCircuitSensorState" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logCircuitSensorAvgValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logCircuitSensorMaxValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logCircuitSensorMinValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logCircuitSensorSignedAvgValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logCircuitSensorSignedMaxValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logCircuitSensorSignedMinValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logCircuitPoleSensorDataAvailable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logCircuitPoleSensorState" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logCircuitPoleSensorAvgValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logCircuitPoleSensorMaxValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logCircuitPoleSensorMinValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logCircuitPoleSensorSignedAvgValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logCircuitPoleSensorSignedMaxValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logCircuitPoleSensorSignedMinValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
}, # members
"description" :
"""A collection of objects providing logging (history of readings) capabilities
about the PDU.""",
}, # group
"measurementsGroup" : {
"nodetype" : "group",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.9.2.3",
"status" : "current",
"members" : {
"measurementsUnitSensorIsAvailable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsUnitSensorState" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsUnitSensorValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsUnitSensorTimeStamp" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsUnitSensorSignedValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsInletSensorIsAvailable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsInletSensorState" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsInletSensorValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsInletSensorTimeStamp" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsInletSensorSignedValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsInletPoleSensorIsAvailable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsInletPoleSensorState" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsInletPoleSensorValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsInletPoleSensorTimeStamp" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsInletPoleSensorSignedValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsOutletSensorIsAvailable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsOutletSensorState" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsOutletSensorValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsOutletSensorTimeStamp" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsOutletSensorSignedValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsOutletPoleSensorIsAvailable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsOutletPoleSensorState" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsOutletPoleSensorValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsOutletPoleSensorTimeStamp" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsOutletPoleSensorSignedValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsOverCurrentProtectorSensorIsAvailable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsOverCurrentProtectorSensorState" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsOverCurrentProtectorSensorValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsOverCurrentProtectorSensorTimeStamp" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsOverCurrentProtectorSensorSignedValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsExternalSensorIsAvailable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsExternalSensorState" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsExternalSensorValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsExternalSensorTimeStamp" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsTransferSwitchSensorIsAvailable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsTransferSwitchSensorState" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsTransferSwitchSensorValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsTransferSwitchSensorTimeStamp" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsTransferSwitchSensorSignedValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsCircuitSensorIsAvailable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsCircuitSensorState" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsCircuitSensorValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsCircuitSensorTimeStamp" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsCircuitSensorSignedValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsCircuitPoleSensorIsAvailable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsCircuitPoleSensorState" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsCircuitPoleSensorValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsCircuitPoleSensorTimeStamp" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsCircuitPoleSensorSignedValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
}, # members
"description" :
"""A collection of objects providing measurements (most recent data) capabilities.
about the PDU.""",
}, # group
"controlGroup" : {
"nodetype" : "group",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.9.2.4",
"status" : "current",
"members" : {
"switchingOperation" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletSwitchingState" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletSwitchingTimeStamp" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchActiveInlet" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchTransferToInlet" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchAlarmOverride" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchLastTransferReason" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"actuatorState" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"rcmState" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletSensorResetValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletSensorResetValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"unitSensorResetValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitSensorResetValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
}, # members
"description" :
"""A collection of objects providing the ability to control various components
of a PDU.""",
}, # group
"trapInformationGroup" : {
"nodetype" : "group",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.9.2.5",
"status" : "current",
"members" : {
"userName" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"targetUser" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"imageVersion" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"roleName" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"oldSensorState" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"pduNumber" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletPoleNumber" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletPoleNumber" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorNumber" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"typeOfSensor" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"smtpMessageRecipients" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"smtpServer" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"errorDescription" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"deviceChangedParameter" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"changedParameterNewValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"lhxSupportEnabled" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"webcamModel" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"webcamConnectionPort" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"agentInetPortNumber" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"peripheralDeviceRomcode" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"peripheralDeviceFirmwareUpdateState" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitNumber" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitPoleNumber" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"phoneNumber" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
}, # members
"description" :
"""A collection of objects providing information in the traps.""",
}, # group
"trapsGroup" : {
"nodetype" : "group",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.9.2.9",
"status" : "current",
"members" : {
"systemStarted" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"systemReset" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"userLogin" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"userLogout" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"userAuthenticationFailure" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"userSessionTimeout" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"userAdded" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"userModified" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"userDeleted" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"roleAdded" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"roleModified" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"roleDeleted" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"deviceUpdateStarted" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"deviceUpdateCompleted" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"userBlocked" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"powerControl" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"userPasswordChanged" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"passwordSettingsChanged" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"firmwareValidationFailed" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logFileCleared" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"bulkConfigurationSaved" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"bulkConfigurationCopied" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletSensorStateChange" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletPoleSensorStateChange" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletSensorStateChange" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletPoleSensorStateChange" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"overCurrentProtectorSensorStateChange" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorStateChange" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"smtpMessageTransmissionFailure" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"ldapError" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"deviceUpdateFailed" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"loadSheddingModeEntered" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"loadSheddingModeExited" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"pingServerEnabled" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"pingServerDisabled" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"serverNotReachable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"serverReachable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"rfCodeTagConnected" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"rfCodeTagDisconnected" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"deviceIdentificationChanged" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"usbSlaveConnected" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"usbSlaveDisconnected" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"lhxSupportChanged" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"userAcceptedRestrictedServiceAgreement" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"userDeclinedRestrictedServiceAgreement" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchSensorStateChange" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"deviceSettingsSaved" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"deviceSettingsRestored" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"webcamInserted" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"webcamRemoved" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletEnabled" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletDisabled" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"serverConnectivityUnrecoverable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"radiusError" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"serverReachabilityError" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletSensorReset" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletSensorReset" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"unitSensorReset" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitSensorReset" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"unknownPeripheralDeviceAttached" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"peripheralDeviceFirmwareUpdate" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"unitSensorStateChange" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitSensorStateChange" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitPoleSensorStateChange" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitAdded" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitDeleted" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"circuitModified" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"powerMeterAdded" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"powerMeterDeleted" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"powerMeterModified" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"smsMessageTransmissionFailure" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
}, # members
"description" :
"""A collection of traps.""",
}, # group
"reliabilityGroup" : {
"nodetype" : "group",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.9.2.10",
"status" : "current",
"members" : {
"reliabilityId" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"reliabilityDataValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"reliabilityDataMaxPossible" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"reliabilityDataWorstValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"reliabilityDataThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"reliabilityDataRawUpperBytes" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"reliabilityDataRawLowerBytes" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"reliabilityDataFlags" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"reliabilityErrorLogId" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"reliabilityErrorLogValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"reliabilityErrorLogThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"reliabilityErrorLogRawUpperBytes" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"reliabilityErrorLogRawLowerBytes" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"reliabilityErrorLogPOH" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"reliabilityErrorLogTime" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"reliabilityDataTableSequenceNumber" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
}, # members
"description" :
"""A collection of objects providing reliability data.""",
}, # group
"ipAddressGroup" : {
"nodetype" : "group",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.9.2.12",
"status" : "deprecated",
"members" : {
"pxIPAddress" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"netmask" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"gateway" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"pxInetAddressType" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"pxInetIPAddress" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"pxInetNetmask" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"pxInetGateway" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
}, # members
"description" :
"""A collection of objects representing configuration data.""",
}, # group
"oldConfigGroup" : {
"nodetype" : "group",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.9.2.13",
"status" : "deprecated",
"members" : {
"outletSequencingDelay" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"unitSensorAccuracy" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"unitSensorTolerance" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletSensorAccuracy" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletSensorTolerance" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletPoleSensorAccuracy" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletPoleSensorTolerance" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletSensorAccuracy" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletSensorTolerance" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletPoleSensorAccuracy" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"outletPoleSensorTolerance" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"overCurrentProtectorSensorAccuracy" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"overCurrentProtectorSensorTolerance" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorAccuracy" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"externalSensorTolerance" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"wireSensorAccuracy" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"wireSensorTolerance" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchSensorAccuracy" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"transferSwitchSensorTolerance" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"wireCount" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"wireLabel" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"wireCapabilities" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"wireSensorLogAvailable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"wireSensorUnits" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"wireSensorDecimalDigits" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"wireSensorResolution" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"wireSensorMaximum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"wireSensorMinimum" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"wireSensorHysteresis" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"wireSensorStateChangeDelay" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"wireSensorLowerCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"wireSensorLowerWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"wireSensorUpperCriticalThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"wireSensorUpperWarningThreshold" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"wireSensorEnabledThresholds" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"wirePowerSource" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletRatedFrequency" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"inletRatedVA" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
}, # members
"description" :
"""A collection of objects representing old (deprecated) configuration data.""",
}, # group
"oldLogGroup" : {
"nodetype" : "group",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.9.2.14",
"status" : "deprecated",
"members" : {
"logWireSensorDataAvailable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logWireSensorState" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logWireSensorAvgValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logWireSensorMaxValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"logWireSensorMinValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
}, # members
"description" :
"""A collection of objects representing old (deprecated) logging data.""",
}, # group
"oldMeasurementsGroup" : {
"nodetype" : "group",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.9.2.15",
"status" : "deprecated",
"members" : {
"measurementsWireSensorIsAvailable" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsWireSensorState" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsWireSensorValue" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"measurementsWireSensorTimeStamp" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
}, # members
"description" :
"""A collection of objects representing old (deprecated) measurement data.""",
}, # group
"oldTrapsGroup" : {
"nodetype" : "group",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.9.2.16",
"status" : "deprecated",
"members" : {
"wireSensorStateChange" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
"pduSensorStateChange" : {
"nodetype" : "member",
"module" : "PDU2-MIB"
},
}, # members
"description" :
"""A collection of objects representing old (deprecated) traps.""",
}, # group
}, # groups
"compliances" : {
"complianceRev1" : {
"nodetype" : "compliance",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.9.1.1",
"status" : "deprecated",
"description" :
"""The requirements for conformance to the PDU2-MIB.""",
"requires" : {
"ipAddressGroup" : {
"nodetype" : "optional",
"module" : "PDU2-MIB",
"description" :
"""The ipAddress group.""",
},
"oldConfigGroup" : {
"nodetype" : "optional",
"module" : "PDU2-MIB",
"description" :
"""old (deprecated) configuration data""",
},
"oldLogGroup" : {
"nodetype" : "optional",
"module" : "PDU2-MIB",
"description" :
"""old (deprecated) logging data""",
},
"oldMeasurementsGroup" : {
"nodetype" : "optional",
"module" : "PDU2-MIB",
"description" :
"""old (deprecated) measurement data""",
},
"oldTrapsGroup" : {
"nodetype" : "optional",
"module" : "PDU2-MIB",
"description" :
"""old (deprecated) traps""",
},
}, # requires
}, # compliance
"complianceRev2" : {
"nodetype" : "compliance",
"moduleName" : "PDU2-MIB",
"oid" : "1.3.6.1.4.1.13742.6.9.1.2",
"status" : "current",
"description" :
"""The requirements for conformance to the PDU2-MIB.""",
"requires" : {
"configGroup" : {
"nodetype" : "optional",
"module" : "PDU2-MIB",
"description" :
"""The configuration group.""",
},
"logGroup" : {
"nodetype" : "optional",
"module" : "PDU2-MIB",
"description" :
"""The log group.""",
},
"measurementsGroup" : {
"nodetype" : "optional",
"module" : "PDU2-MIB",
"description" :
"""The measurements group.""",
},
"controlGroup" : {
"nodetype" : "optional",
"module" : "PDU2-MIB",
"description" :
"""The control group.""",
},
"trapInformationGroup" : {
"nodetype" : "optional",
"module" : "PDU2-MIB",
"description" :
"""The information group.""",
},
"trapsGroup" : {
"nodetype" : "optional",
"module" : "PDU2-MIB",
"description" :
"""The information group.""",
},
"reliabilityGroup" : {
"nodetype" : "optional",
"module" : "PDU2-MIB",
"description" :
"""The reliability group.""",
},
}, # requires
"refinements" : {
"powerMeterPhaseCTRating" : {
"module" : "PDU2-MIB",
"access" : "readonly",
"description" :
"""configuration is not required""",
},
"powerMeterNeutralCTRating" : {
"module" : "PDU2-MIB",
"access" : "readonly",
"description" :
"""configuration is not required""",
},
"powerMeterEarthCTRating" : {
"module" : "PDU2-MIB",
"access" : "readonly",
"description" :
"""configuration is not required""",
},
"circuitName" : {
"module" : "PDU2-MIB",
"access" : "readonly",
"description" :
"""configuration is not required""",
},
"circuitRatedCurrent" : {
"module" : "PDU2-MIB",
"access" : "readonly",
"description" :
"""configuration is not required""",
},
"circuitPoleCTNumber" : {
"module" : "PDU2-MIB",
"access" : "readonly",
"description" :
"""configuration is not required""",
},
"circuitPolePhase" : {
"module" : "PDU2-MIB",
"access" : "readonly",
"description" :
"""configuration is not required""",
},
"circuitCTRating" : {
"module" : "PDU2-MIB",
"access" : "readonly",
"description" :
"""configuration is not required""",
},
}, # refinements
}, # compliance
}, # compliances
}
| sigmunau/nav | python/nav/smidumps/pdu2_mib.py | Python | gpl-2.0 | 809,850 |
import re
import logging
import time
import random
import string
import aexpect
from autotest.client.shared import error
from virttest import data_dir
from virttest import utils_misc
from virttest import storage
from virttest import arch
from virttest import env_process
@error.context_aware
def run(test, params, env):
"""
Test hotplug of PCI devices and check the status in guest.
1 Boot up a guest
2 Hotplug virtio disk to the guest. Record the id and partition name of
the disk in a list.
3 Random choice a disk in the list. Unplug the disk and check the
partition status.
4 Hotpulg the disk back to guest with the same monitor cmdline and same
id which is record in step 2.
5 Check the partition status in guest. And confirm the disk with dd cmd
6 Repeat step 3 to 5 for N times
:param test: KVM test object.
:param params: Dictionary with the test parameters.
:param env: Dictionary with test environment.
"""
def prepare_image_params(params):
pci_num = int(params['pci_num'])
for i in xrange(pci_num):
image_name = '%s_%s' % ('stg', i)
params['images'] = ' '.join([params['images'], image_name])
image_image_name = '%s_%s' % ('image_name', image_name)
params[image_image_name] = '%s_%s' % ('storage', i)
image_image_format = '%s_%s' % ('image_format', image_name)
params[image_image_format] = params.get('image_format_extra', 'qcow2')
image_image_size = '%s_%s' % ('image_size', image_name)
params[image_image_size] = params.get('image_size_extra', '128K')
return params
def find_new_device(check_cmd, device_string, chk_timeout=5.0):
end_time = time.time() + chk_timeout
idx = ("wmic" in check_cmd and [0] or [-1])[0]
while time.time() < end_time:
new_line = session.cmd_output(check_cmd)
for line in re.split("\n+", new_line.strip()):
dev_name = re.split("\s+", line.strip())[idx]
if dev_name not in device_string:
return dev_name
time.sleep(0.1)
return None
def find_del_device(check_cmd, device_string, chk_timeout=5.0):
end_time = time.time() + chk_timeout
idx = ("wmic" in check_cmd and [0] or [-1])[0]
while time.time() < end_time:
new_line = session.cmd_output(check_cmd)
for line in re.split("\n+", device_string.strip()):
dev_name = re.split("\s+", line.strip())[idx]
if dev_name not in new_line:
return dev_name
time.sleep(0.1)
return None
# Select an image file
def find_image(pci_num):
image_params = params.object_params("%s" % img_list[pci_num + 1])
o = storage.get_image_filename(image_params, data_dir.get_data_dir())
return o
def pci_add_block(pci_num, queues, pci_id):
image_filename = find_image(pci_num)
pci_add_cmd = ("pci_add pci_addr=auto storage file=%s,if=%s" %
(image_filename, pci_model))
return pci_add(pci_add_cmd)
def pci_add(pci_add_cmd):
guest_devices = session.cmd_output(chk_cmd)
error.context("Adding pci device with command 'pci_add'")
add_output = vm.monitor.send_args_cmd(pci_add_cmd, convert=False)
guest_device = find_new_device(chk_cmd, guest_devices)
pci_info.append(['', '', add_output, pci_model, guest_device])
if "OK domain" not in add_output:
raise error.TestFail("Add PCI device failed. "
"Monitor command is: %s, Output: %r" %
(pci_add_cmd, add_output))
return vm.monitor.info("pci")
def is_supported_device(dev):
# Probe qemu to verify what is the supported syntax for PCI hotplug
cmd_output = vm.monitor.human_monitor_cmd("?")
if len(re.findall("\ndevice_add", cmd_output)) > 0:
cmd_type = "device_add"
elif len(re.findall("\npci_add", cmd_output)) > 0:
cmd_type = "pci_add"
else:
raise error.TestError("Unknow version of qemu")
# Probe qemu for a list of supported devices
probe_output = vm.monitor.human_monitor_cmd("%s ?" % cmd_type)
devices_supported = [j.strip('"') for j in
re.findall('\"[a-z|0-9|\-|\_|\,|\.]*\"',
probe_output, re.MULTILINE)]
logging.debug("QEMU reported the following supported devices for "
"PCI hotplug: %s", devices_supported)
return (dev in devices_supported)
def verify_supported_device(dev):
if not is_supported_device(dev):
raise error.TestError("%s doesn't support device: %s" %
(cmd_type, dev))
def device_add_block(pci_num, queues=1, pci_id=None):
if pci_id is not None:
device_id = pci_type + "-" + pci_id
else:
device_id = pci_type + "-" + utils_misc.generate_random_id()
pci_info.append([device_id, device_id])
image_format = params.get("image_format_%s" % img_list[pci_num + 1])
if not image_format:
image_format = params.get("image_format", "qcow2")
image_filename = find_image(pci_num)
pci_model = params.get("pci_model")
controller_model = None
if pci_model == "virtio":
pci_model = "virtio-blk-pci"
if pci_model == "scsi":
pci_model = "scsi-disk"
if arch.ARCH in ('ppc64', 'ppc64le'):
controller_model = "spapr-vscsi"
else:
controller_model = "lsi53c895a"
verify_supported_device(controller_model)
controller_id = "controller-" + device_id
controller_add_cmd = ("device_add %s,id=%s" %
(controller_model, controller_id))
error.context("Adding SCSI controller.")
vm.monitor.send_args_cmd(controller_add_cmd)
verify_supported_device(pci_model)
if drive_cmd_type == "drive_add":
driver_add_cmd = ("%s auto file=%s,if=none,format=%s,id=%s" %
(drive_cmd_type, image_filename, image_format,
pci_info[pci_num][0]))
elif drive_cmd_type == "__com.redhat_drive_add":
driver_add_cmd = ("%s file=%s,format=%s,id=%s" %
(drive_cmd_type, image_filename, image_format,
pci_info[pci_num][0]))
# add driver.
error.context("Adding driver.")
vm.monitor.send_args_cmd(driver_add_cmd, convert=False)
pci_add_cmd = ("device_add id=%s,driver=%s,drive=%s" %
(pci_info[pci_num][1],
pci_model,
pci_info[pci_num][0])
)
return device_add(pci_num, pci_add_cmd, pci_id=pci_id)
def device_add(pci_num, pci_add_cmd, pci_id=None):
error.context("Adding pci device with command 'device_add'")
guest_devices = session.cmd_output(chk_cmd)
if vm.monitor.protocol == 'qmp':
add_output = vm.monitor.send_args_cmd(pci_add_cmd)
else:
add_output = vm.monitor.send_args_cmd(pci_add_cmd, convert=False)
guest_device = find_new_device(chk_cmd, guest_devices)
if pci_id is None:
pci_info[pci_num].append(add_output)
pci_info[pci_num].append(pci_model)
pci_info[pci_num].append(guest_device)
after_add = vm.monitor.info("pci")
if pci_info[pci_num][1] not in str(after_add):
logging.error("Could not find matched id in monitor:"
" %s" % pci_info[pci_num][1])
raise error.TestFail("Add device failed. Monitor command is: %s"
". Output: %r" % (pci_add_cmd, add_output))
return after_add
# Hot add a pci device
def add_device(pci_num, queues=1, pci_id=None):
info_pci_ref = vm.monitor.info("pci")
reference = session.cmd_output(reference_cmd)
try:
# get function for adding device.
add_fuction = local_functions["%s_%s" % (cmd_type, pci_type)]
except Exception:
raise error.TestError("No function for adding " +
"'%s' dev " % pci_type +
"with '%s'" % cmd_type)
after_add = None
if add_fuction:
# Do add pci device.
after_add = add_fuction(pci_num, queues, pci_id)
try:
# Define a helper function to compare the output
def _new_shown():
o = session.cmd_output(reference_cmd)
return o != reference
# Define a helper function to catch PCI device string
def _find_pci():
output = session.cmd_output(params.get("find_pci_cmd"))
output = map(string.strip, output.splitlines())
ref = map(string.strip, reference.splitlines())
output = [_ for _ in output if _ not in ref]
output = "\n".join(output)
if re.search(params.get("match_string"), output, re.I):
return True
return False
error.context("Start checking new added device")
# Compare the output of 'info pci'
if after_add == info_pci_ref:
raise error.TestFail("No new PCI device shown after "
"executing monitor command: 'info pci'")
secs = int(params.get("wait_secs_for_hook_up"))
if not utils_misc.wait_for(_new_shown, test_timeout, secs, 3):
raise error.TestFail("No new device shown in output of" +
"command executed inside the " +
"guest: %s" % reference_cmd)
if not utils_misc.wait_for(_find_pci, test_timeout, 3, 3):
raise error.TestFail("PCI %s %s " % (pci_model, pci_type) +
"device not found in guest. Command " +
"was: %s" % params.get("find_pci_cmd"))
# Test the newly added device
try:
session.cmd(params.get("pci_test_cmd") % (pci_num + 1))
except aexpect.ShellError, e:
raise error.TestFail("Check for %s device failed" % pci_type +
"after PCI hotplug." +
"Output: %r" % e.output)
except Exception:
pci_del(pci_num, ignore_failure=True)
raise
# Hot delete a pci device
def pci_del(pci_num, ignore_failure=False):
def _device_removed():
after_del = vm.monitor.info("pci")
return after_del != before_del
before_del = vm.monitor.info("pci")
if cmd_type == "pci_add":
slot_id = int(pci_info[pci_num][2].split(",")[2].split()[1])
cmd = "pci_del pci_addr=%s" % hex(slot_id)
vm.monitor.send_args_cmd(cmd, convert=False)
elif cmd_type == "device_add":
cmd = "device_del id=%s" % pci_info[pci_num][1]
vm.monitor.send_args_cmd(cmd)
if (not utils_misc.wait_for(_device_removed, test_timeout, 0, 1) and
not ignore_failure):
raise error.TestFail("Failed to hot remove PCI device: %s. "
"Monitor command: %s" %
(pci_info[pci_num][3], cmd))
params = prepare_image_params(params)
env_process.process_images(env_process.preprocess_image, test, params)
vm = env.get_vm(params["main_vm"])
vm.verify_alive()
timeout = int(params.get("login_timeout", 360))
session = vm.wait_for_login(timeout=timeout)
test_timeout = int(params.get("hotplug_timeout", 360))
reference_cmd = params["reference_cmd"]
# Test if it is nic or block
pci_type = params["pci_type"]
pci_model = params["pci_model"]
# Modprobe the module if specified in config file
module = params.get("modprobe_module")
if module:
session.cmd("modprobe %s" % module)
# check monitor type
qemu_binary = utils_misc.get_qemu_binary(params)
# Probe qemu to verify what is the supported syntax for PCI hotplug
if vm.monitor.protocol == 'qmp':
cmd_output = vm.monitor.info("commands")
else:
cmd_output = vm.monitor.human_monitor_cmd("help", debug=False)
cmd_type = utils_misc.find_substring(str(cmd_output), "device_add",
"pci_add")
if not cmd_type:
raise error.TestError("Could find a suitable method for hotplugging"
" device in this version of qemu")
# Determine syntax of drive hotplug
# __com.redhat_drive_add == qemu-kvm-0.12 on RHEL 6
# drive_add == qemu-kvm-0.13 onwards
drive_cmd_type = utils_misc.find_substring(str(cmd_output),
"__com.redhat_drive_add",
"drive_add")
if not drive_cmd_type:
raise error.TestError("Unknow version of qemu")
local_functions = locals()
pci_num_range = int(params.get("pci_num"))
rp_times = int(params.get("repeat_times"))
img_list = params.get("images").split()
chk_cmd = params.get("guest_check_cmd")
mark_cmd = params.get("mark_cmd")
offset = params.get("offset")
confirm_cmd = params.get("confirm_cmd")
pci_info = []
# Add block device into guest
for pci_num in xrange(pci_num_range):
error.context("Prepare the %d removable pci device" % pci_num,
logging.info)
add_device(pci_num)
if pci_info[pci_num][4] is not None:
partition = pci_info[pci_num][4]
cmd = mark_cmd % (partition, partition, offset)
session.cmd(cmd)
else:
raise error.TestError("Device not init in guest")
for j in range(rp_times):
# pci_info is a list of list.
# each element 'i' has 4 members:
# pci_info[i][0] == device drive id, only used for device_add
# pci_info[i][1] == device id, only used for device_add
# pci_info[i][2] == output of device add command
# pci_info[i][3] == device module name.
# pci_info[i][4] == partition id in guest
pci_num = random.randint(0, len(pci_info) - 1)
error.context("start unplug pci device, repeat %d" % j, logging.info)
guest_devices = session.cmd_output(chk_cmd)
pci_del(pci_num)
device_del = find_del_device(chk_cmd, guest_devices)
if device_del != pci_info[pci_num][4]:
raise error.TestFail("Device is not deleted in guest.")
error.context("Start plug pci device, repeat %d" % j, logging.info)
guest_devices = session.cmd_output(chk_cmd)
add_device(pci_num, pci_id=pci_info[pci_num][0])
device_del = find_new_device(chk_cmd, guest_devices)
if device_del != pci_info[pci_num][4]:
raise error.TestFail("Device partition changed from %s to %s" %
(pci_info[pci_num][4], device_del))
cmd = confirm_cmd % (pci_info[pci_num][4], offset)
confirm_info = session.cmd_output(cmd)
if device_del not in confirm_info:
raise error.TestFail("Can not find partition tag in Guest: %s" %
confirm_info)
| tolimit/tp-qemu | qemu/tests/pci_hotplug_check.py | Python | gpl-2.0 | 15,817 |
import contextlib
import copy
import inspect
import pickle
import re
import sys
import types
import unittest
import warnings
from test import support
from test.support.script_helper import assert_python_ok
class AsyncYieldFrom:
def __init__(self, obj):
self.obj = obj
def __await__(self):
yield from self.obj
class AsyncYield:
def __init__(self, value):
self.value = value
def __await__(self):
yield self.value
def run_async(coro):
assert coro.__class__ in {types.GeneratorType, types.CoroutineType}
buffer = []
result = None
while True:
try:
buffer.append(coro.send(None))
except StopIteration as ex:
result = ex.args[0] if ex.args else None
break
return buffer, result
def run_async__await__(coro):
assert coro.__class__ is types.CoroutineType
aw = coro.__await__()
buffer = []
result = None
i = 0
while True:
try:
if i % 2:
buffer.append(next(aw))
else:
buffer.append(aw.send(None))
i += 1
except StopIteration as ex:
result = ex.args[0] if ex.args else None
break
return buffer, result
@contextlib.contextmanager
def silence_coro_gc():
with warnings.catch_warnings():
warnings.simplefilter("ignore")
yield
support.gc_collect()
class AsyncBadSyntaxTest(unittest.TestCase):
def test_badsyntax_1(self):
samples = [
"""def foo():
await something()
""",
"""await something()""",
"""async def foo():
yield from []
""",
"""async def foo():
await await fut
""",
"""async def foo(a=await something()):
pass
""",
"""async def foo(a:await something()):
pass
""",
"""async def foo():
def bar():
[i async for i in els]
""",
"""async def foo():
def bar():
[await i for i in els]
""",
"""async def foo():
def bar():
[i for i in els
async for b in els]
""",
"""async def foo():
def bar():
[i for i in els
for c in b
async for b in els]
""",
"""async def foo():
def bar():
[i for i in els
async for b in els
for c in b]
""",
"""async def foo():
def bar():
[i for i in els
for b in await els]
""",
"""async def foo():
def bar():
[i for i in els
for b in els
if await b]
""",
"""async def foo():
def bar():
[i for i in await els]
""",
"""async def foo():
def bar():
[i for i in els if await i]
""",
"""def bar():
[i async for i in els]
""",
"""def bar():
{i: i async for i in els}
""",
"""def bar():
{i async for i in els}
""",
"""def bar():
[await i for i in els]
""",
"""def bar():
[i for i in els
async for b in els]
""",
"""def bar():
[i for i in els
for c in b
async for b in els]
""",
"""def bar():
[i for i in els
async for b in els
for c in b]
""",
"""def bar():
[i for i in els
for b in await els]
""",
"""def bar():
[i for i in els
for b in els
if await b]
""",
"""def bar():
[i for i in await els]
""",
"""def bar():
[i for i in els if await i]
""",
"""async def foo():
await
""",
"""async def foo():
def bar(): pass
await = 1
""",
"""async def foo():
def bar(): pass
await = 1
""",
"""async def foo():
def bar(): pass
if 1:
await = 1
""",
"""def foo():
async def bar(): pass
if 1:
await a
""",
"""def foo():
async def bar(): pass
await a
""",
"""def foo():
def baz(): pass
async def bar(): pass
await a
""",
"""def foo():
def baz(): pass
# 456
async def bar(): pass
# 123
await a
""",
"""async def foo():
def baz(): pass
# 456
async def bar(): pass
# 123
await = 2
""",
"""def foo():
def baz(): pass
async def bar(): pass
await a
""",
"""async def foo():
def baz(): pass
async def bar(): pass
await = 2
""",
"""async def foo():
def async(): pass
""",
"""async def foo():
def await(): pass
""",
"""async def foo():
def bar():
await
""",
"""async def foo():
return lambda async: await
""",
"""async def foo():
return lambda a: await
""",
"""await a()""",
"""async def foo(a=await b):
pass
""",
"""async def foo(a:await b):
pass
""",
"""def baz():
async def foo(a=await b):
pass
""",
"""async def foo(async):
pass
""",
"""async def foo():
def bar():
def baz():
async = 1
""",
"""async def foo():
def bar():
def baz():
pass
async = 1
""",
"""def foo():
async def bar():
async def baz():
pass
def baz():
42
async = 1
""",
"""async def foo():
def bar():
def baz():
pass\nawait foo()
""",
"""def foo():
def bar():
async def baz():
pass\nawait foo()
""",
"""async def foo(await):
pass
""",
"""def foo():
async def bar(): pass
await a
""",
"""def foo():
async def bar():
pass\nawait a
""",
"""def foo():
async for i in arange(2):
pass
""",
"""def foo():
async with resource:
pass
""",
"""async with resource:
pass
""",
"""async for i in arange(2):
pass
""",
]
for code in samples:
with self.subTest(code=code), self.assertRaises(SyntaxError):
compile(code, "<test>", "exec")
def test_badsyntax_2(self):
samples = [
"""def foo():
await = 1
""",
"""class Bar:
def async(): pass
""",
"""class Bar:
async = 1
""",
"""class async:
pass
""",
"""class await:
pass
""",
"""import math as await""",
"""def async():
pass""",
"""def foo(*, await=1):
pass"""
"""async = 1""",
"""print(await=1)"""
]
for code in samples:
with self.subTest(code=code), self.assertRaises(SyntaxError):
compile(code, "<test>", "exec")
def test_badsyntax_3(self):
with self.assertRaises(SyntaxError):
compile("async = 1", "<test>", "exec")
def test_badsyntax_4(self):
samples = [
'''def foo(await):
async def foo(): pass
async def foo():
pass
return await + 1
''',
'''def foo(await):
async def foo(): pass
async def foo(): pass
return await + 1
''',
'''def foo(await):
async def foo(): pass
async def foo(): pass
return await + 1
''',
'''def foo(await):
"""spam"""
async def foo(): \
pass
# 123
async def foo(): pass
# 456
return await + 1
''',
'''def foo(await):
def foo(): pass
def foo(): pass
async def bar(): return await_
await_ = await
try:
bar().send(None)
except StopIteration as ex:
return ex.args[0] + 1
'''
]
for code in samples:
with self.subTest(code=code), self.assertRaises(SyntaxError):
compile(code, "<test>", "exec")
class TokenizerRegrTest(unittest.TestCase):
def test_oneline_defs(self):
buf = []
for i in range(500):
buf.append('def i{i}(): return {i}'.format(i=i))
buf = '\n'.join(buf)
# Test that 500 consequent, one-line defs is OK
ns = {}
exec(buf, ns, ns)
self.assertEqual(ns['i499'](), 499)
# Test that 500 consequent, one-line defs *and*
# one 'async def' following them is OK
buf += '\nasync def foo():\n return'
ns = {}
exec(buf, ns, ns)
self.assertEqual(ns['i499'](), 499)
self.assertTrue(inspect.iscoroutinefunction(ns['foo']))
class CoroutineTest(unittest.TestCase):
def test_gen_1(self):
def gen(): yield
self.assertFalse(hasattr(gen, '__await__'))
def test_func_1(self):
async def foo():
return 10
f = foo()
self.assertIsInstance(f, types.CoroutineType)
self.assertTrue(bool(foo.__code__.co_flags & inspect.CO_COROUTINE))
self.assertFalse(bool(foo.__code__.co_flags & inspect.CO_GENERATOR))
self.assertTrue(bool(f.cr_code.co_flags & inspect.CO_COROUTINE))
self.assertFalse(bool(f.cr_code.co_flags & inspect.CO_GENERATOR))
self.assertEqual(run_async(f), ([], 10))
self.assertEqual(run_async__await__(foo()), ([], 10))
def bar(): pass
self.assertFalse(bool(bar.__code__.co_flags & inspect.CO_COROUTINE))
def test_func_2(self):
async def foo():
raise StopIteration
with self.assertRaisesRegex(
RuntimeError, "coroutine raised StopIteration"):
run_async(foo())
def test_func_3(self):
async def foo():
raise StopIteration
coro = foo()
self.assertRegex(repr(coro), '^<coroutine object.* at 0x.*>$')
coro.close()
def test_func_4(self):
async def foo():
raise StopIteration
coro = foo()
check = lambda: self.assertRaisesRegex(
TypeError, "'coroutine' object is not iterable")
with check():
list(coro)
with check():
tuple(coro)
with check():
sum(coro)
with check():
iter(coro)
with check():
for i in coro:
pass
with check():
[i for i in coro]
coro.close()
def test_func_5(self):
@types.coroutine
def bar():
yield 1
async def foo():
await bar()
check = lambda: self.assertRaisesRegex(
TypeError, "'coroutine' object is not iterable")
coro = foo()
with check():
for el in coro:
pass
coro.close()
# the following should pass without an error
for el in bar():
self.assertEqual(el, 1)
self.assertEqual([el for el in bar()], [1])
self.assertEqual(tuple(bar()), (1,))
self.assertEqual(next(iter(bar())), 1)
def test_func_6(self):
@types.coroutine
def bar():
yield 1
yield 2
async def foo():
await bar()
f = foo()
self.assertEqual(f.send(None), 1)
self.assertEqual(f.send(None), 2)
with self.assertRaises(StopIteration):
f.send(None)
def test_func_7(self):
async def bar():
return 10
coro = bar()
def foo():
yield from coro
with self.assertRaisesRegex(
TypeError,
"cannot 'yield from' a coroutine object in "
"a non-coroutine generator"):
list(foo())
coro.close()
def test_func_8(self):
@types.coroutine
def bar():
return (yield from coro)
async def foo():
return 'spam'
coro = foo()
self.assertEqual(run_async(bar()), ([], 'spam'))
coro.close()
def test_func_9(self):
async def foo():
pass
with self.assertWarnsRegex(
RuntimeWarning,
r"coroutine '.*test_func_9.*foo' was never awaited"):
foo()
support.gc_collect()
with self.assertWarnsRegex(
RuntimeWarning,
r"coroutine '.*test_func_9.*foo' was never awaited"):
with self.assertRaises(TypeError):
# See bpo-32703.
for _ in foo():
pass
support.gc_collect()
def test_func_10(self):
N = 0
@types.coroutine
def gen():
nonlocal N
try:
a = yield
yield (a ** 2)
except ZeroDivisionError:
N += 100
raise
finally:
N += 1
async def foo():
await gen()
coro = foo()
aw = coro.__await__()
self.assertIs(aw, iter(aw))
next(aw)
self.assertEqual(aw.send(10), 100)
self.assertEqual(N, 0)
aw.close()
self.assertEqual(N, 1)
coro = foo()
aw = coro.__await__()
next(aw)
with self.assertRaises(ZeroDivisionError):
aw.throw(ZeroDivisionError, None, None)
self.assertEqual(N, 102)
def test_func_11(self):
async def func(): pass
coro = func()
# Test that PyCoro_Type and _PyCoroWrapper_Type types were properly
# initialized
self.assertIn('__await__', dir(coro))
self.assertIn('__iter__', dir(coro.__await__()))
self.assertIn('coroutine_wrapper', repr(coro.__await__()))
coro.close() # avoid RuntimeWarning
def test_func_12(self):
async def g():
i = me.send(None)
await foo
me = g()
with self.assertRaisesRegex(ValueError,
"coroutine already executing"):
me.send(None)
def test_func_13(self):
async def g():
pass
coro = g()
with self.assertRaisesRegex(
TypeError,
"can't send non-None value to a just-started coroutine"):
coro.send('spam')
coro.close()
def test_func_14(self):
@types.coroutine
def gen():
yield
async def coro():
try:
await gen()
except GeneratorExit:
await gen()
c = coro()
c.send(None)
with self.assertRaisesRegex(RuntimeError,
"coroutine ignored GeneratorExit"):
c.close()
def test_func_15(self):
# See http://bugs.python.org/issue25887 for details
async def spammer():
return 'spam'
async def reader(coro):
return await coro
spammer_coro = spammer()
with self.assertRaisesRegex(StopIteration, 'spam'):
reader(spammer_coro).send(None)
with self.assertRaisesRegex(RuntimeError,
'cannot reuse already awaited coroutine'):
reader(spammer_coro).send(None)
def test_func_16(self):
# See http://bugs.python.org/issue25887 for details
@types.coroutine
def nop():
yield
async def send():
await nop()
return 'spam'
async def read(coro):
await nop()
return await coro
spammer = send()
reader = read(spammer)
reader.send(None)
reader.send(None)
with self.assertRaisesRegex(Exception, 'ham'):
reader.throw(Exception('ham'))
reader = read(spammer)
reader.send(None)
with self.assertRaisesRegex(RuntimeError,
'cannot reuse already awaited coroutine'):
reader.send(None)
with self.assertRaisesRegex(RuntimeError,
'cannot reuse already awaited coroutine'):
reader.throw(Exception('wat'))
def test_func_17(self):
# See http://bugs.python.org/issue25887 for details
async def coroutine():
return 'spam'
coro = coroutine()
with self.assertRaisesRegex(StopIteration, 'spam'):
coro.send(None)
with self.assertRaisesRegex(RuntimeError,
'cannot reuse already awaited coroutine'):
coro.send(None)
with self.assertRaisesRegex(RuntimeError,
'cannot reuse already awaited coroutine'):
coro.throw(Exception('wat'))
# Closing a coroutine shouldn't raise any exception even if it's
# already closed/exhausted (similar to generators)
coro.close()
coro.close()
def test_func_18(self):
# See http://bugs.python.org/issue25887 for details
async def coroutine():
return 'spam'
coro = coroutine()
await_iter = coro.__await__()
it = iter(await_iter)
with self.assertRaisesRegex(StopIteration, 'spam'):
it.send(None)
with self.assertRaisesRegex(RuntimeError,
'cannot reuse already awaited coroutine'):
it.send(None)
with self.assertRaisesRegex(RuntimeError,
'cannot reuse already awaited coroutine'):
# Although the iterator protocol requires iterators to
# raise another StopIteration here, we don't want to do
# that. In this particular case, the iterator will raise
# a RuntimeError, so that 'yield from' and 'await'
# expressions will trigger the error, instead of silently
# ignoring the call.
next(it)
with self.assertRaisesRegex(RuntimeError,
'cannot reuse already awaited coroutine'):
it.throw(Exception('wat'))
with self.assertRaisesRegex(RuntimeError,
'cannot reuse already awaited coroutine'):
it.throw(Exception('wat'))
# Closing a coroutine shouldn't raise any exception even if it's
# already closed/exhausted (similar to generators)
it.close()
it.close()
def test_func_19(self):
CHK = 0
@types.coroutine
def foo():
nonlocal CHK
yield
try:
yield
except GeneratorExit:
CHK += 1
async def coroutine():
await foo()
coro = coroutine()
coro.send(None)
coro.send(None)
self.assertEqual(CHK, 0)
coro.close()
self.assertEqual(CHK, 1)
for _ in range(3):
# Closing a coroutine shouldn't raise any exception even if it's
# already closed/exhausted (similar to generators)
coro.close()
self.assertEqual(CHK, 1)
def test_coro_wrapper_send_tuple(self):
async def foo():
return (10,)
result = run_async__await__(foo())
self.assertEqual(result, ([], (10,)))
def test_coro_wrapper_send_stop_iterator(self):
async def foo():
return StopIteration(10)
result = run_async__await__(foo())
self.assertIsInstance(result[1], StopIteration)
self.assertEqual(result[1].value, 10)
def test_cr_await(self):
@types.coroutine
def a():
self.assertEqual(inspect.getcoroutinestate(coro_b), inspect.CORO_RUNNING)
self.assertIsNone(coro_b.cr_await)
yield
self.assertEqual(inspect.getcoroutinestate(coro_b), inspect.CORO_RUNNING)
self.assertIsNone(coro_b.cr_await)
async def c():
await a()
async def b():
self.assertIsNone(coro_b.cr_await)
await c()
self.assertIsNone(coro_b.cr_await)
coro_b = b()
self.assertEqual(inspect.getcoroutinestate(coro_b), inspect.CORO_CREATED)
self.assertIsNone(coro_b.cr_await)
coro_b.send(None)
self.assertEqual(inspect.getcoroutinestate(coro_b), inspect.CORO_SUSPENDED)
self.assertEqual(coro_b.cr_await.cr_await.gi_code.co_name, 'a')
with self.assertRaises(StopIteration):
coro_b.send(None) # complete coroutine
self.assertEqual(inspect.getcoroutinestate(coro_b), inspect.CORO_CLOSED)
self.assertIsNone(coro_b.cr_await)
def test_corotype_1(self):
ct = types.CoroutineType
self.assertIn('into coroutine', ct.send.__doc__)
self.assertIn('inside coroutine', ct.close.__doc__)
self.assertIn('in coroutine', ct.throw.__doc__)
self.assertIn('of the coroutine', ct.__dict__['__name__'].__doc__)
self.assertIn('of the coroutine', ct.__dict__['__qualname__'].__doc__)
self.assertEqual(ct.__name__, 'coroutine')
async def f(): pass
c = f()
self.assertIn('coroutine object', repr(c))
c.close()
def test_await_1(self):
async def foo():
await 1
with self.assertRaisesRegex(TypeError, "object int can.t.*await"):
run_async(foo())
def test_await_2(self):
async def foo():
await []
with self.assertRaisesRegex(TypeError, "object list can.t.*await"):
run_async(foo())
def test_await_3(self):
async def foo():
await AsyncYieldFrom([1, 2, 3])
self.assertEqual(run_async(foo()), ([1, 2, 3], None))
self.assertEqual(run_async__await__(foo()), ([1, 2, 3], None))
def test_await_4(self):
async def bar():
return 42
async def foo():
return await bar()
self.assertEqual(run_async(foo()), ([], 42))
def test_await_5(self):
class Awaitable:
def __await__(self):
return
async def foo():
return (await Awaitable())
with self.assertRaisesRegex(
TypeError, "__await__.*returned non-iterator of type"):
run_async(foo())
def test_await_6(self):
class Awaitable:
def __await__(self):
return iter([52])
async def foo():
return (await Awaitable())
self.assertEqual(run_async(foo()), ([52], None))
def test_await_7(self):
class Awaitable:
def __await__(self):
yield 42
return 100
async def foo():
return (await Awaitable())
self.assertEqual(run_async(foo()), ([42], 100))
def test_await_8(self):
class Awaitable:
pass
async def foo(): return await Awaitable()
with self.assertRaisesRegex(
TypeError, "object Awaitable can't be used in 'await' expression"):
run_async(foo())
def test_await_9(self):
def wrap():
return bar
async def bar():
return 42
async def foo():
db = {'b': lambda: wrap}
class DB:
b = wrap
return (await bar() + await wrap()() + await db['b']()()() +
await bar() * 1000 + await DB.b()())
async def foo2():
return -await bar()
self.assertEqual(run_async(foo()), ([], 42168))
self.assertEqual(run_async(foo2()), ([], -42))
def test_await_10(self):
async def baz():
return 42
async def bar():
return baz()
async def foo():
return await (await bar())
self.assertEqual(run_async(foo()), ([], 42))
def test_await_11(self):
def ident(val):
return val
async def bar():
return 'spam'
async def foo():
return ident(val=await bar())
async def foo2():
return await bar(), 'ham'
self.assertEqual(run_async(foo2()), ([], ('spam', 'ham')))
def test_await_12(self):
async def coro():
return 'spam'
c = coro()
class Awaitable:
def __await__(self):
return c
async def foo():
return await Awaitable()
with self.assertRaisesRegex(
TypeError, r"__await__\(\) returned a coroutine"):
run_async(foo())
c.close()
def test_await_13(self):
class Awaitable:
def __await__(self):
return self
async def foo():
return await Awaitable()
with self.assertRaisesRegex(
TypeError, "__await__.*returned non-iterator of type"):
run_async(foo())
def test_await_14(self):
class Wrapper:
# Forces the interpreter to use CoroutineType.__await__
def __init__(self, coro):
assert coro.__class__ is types.CoroutineType
self.coro = coro
def __await__(self):
return self.coro.__await__()
class FutureLike:
def __await__(self):
return (yield)
class Marker(Exception):
pass
async def coro1():
try:
return await FutureLike()
except ZeroDivisionError:
raise Marker
async def coro2():
return await Wrapper(coro1())
c = coro2()
c.send(None)
with self.assertRaisesRegex(StopIteration, 'spam'):
c.send('spam')
c = coro2()
c.send(None)
with self.assertRaises(Marker):
c.throw(ZeroDivisionError)
def test_await_15(self):
@types.coroutine
def nop():
yield
async def coroutine():
await nop()
async def waiter(coro):
await coro
coro = coroutine()
coro.send(None)
with self.assertRaisesRegex(RuntimeError,
"coroutine is being awaited already"):
waiter(coro).send(None)
def test_await_16(self):
# See https://bugs.python.org/issue29600 for details.
async def f():
return ValueError()
async def g():
try:
raise KeyError
except:
return await f()
_, result = run_async(g())
self.assertIsNone(result.__context__)
def test_with_1(self):
class Manager:
def __init__(self, name):
self.name = name
async def __aenter__(self):
await AsyncYieldFrom(['enter-1-' + self.name,
'enter-2-' + self.name])
return self
async def __aexit__(self, *args):
await AsyncYieldFrom(['exit-1-' + self.name,
'exit-2-' + self.name])
if self.name == 'B':
return True
async def foo():
async with Manager("A") as a, Manager("B") as b:
await AsyncYieldFrom([('managers', a.name, b.name)])
1/0
f = foo()
result, _ = run_async(f)
self.assertEqual(
result, ['enter-1-A', 'enter-2-A', 'enter-1-B', 'enter-2-B',
('managers', 'A', 'B'),
'exit-1-B', 'exit-2-B', 'exit-1-A', 'exit-2-A']
)
async def foo():
async with Manager("A") as a, Manager("C") as c:
await AsyncYieldFrom([('managers', a.name, c.name)])
1/0
with self.assertRaises(ZeroDivisionError):
run_async(foo())
def test_with_2(self):
class CM:
def __aenter__(self):
pass
async def foo():
async with CM():
pass
with self.assertRaisesRegex(AttributeError, '__aexit__'):
run_async(foo())
def test_with_3(self):
class CM:
def __aexit__(self):
pass
async def foo():
async with CM():
pass
with self.assertRaisesRegex(AttributeError, '__aenter__'):
run_async(foo())
def test_with_4(self):
class CM:
def __enter__(self):
pass
def __exit__(self):
pass
async def foo():
async with CM():
pass
with self.assertRaisesRegex(AttributeError, '__aexit__'):
run_async(foo())
def test_with_5(self):
# While this test doesn't make a lot of sense,
# it's a regression test for an early bug with opcodes
# generation
class CM:
async def __aenter__(self):
return self
async def __aexit__(self, *exc):
pass
async def func():
async with CM():
assert (1, ) == 1
with self.assertRaises(AssertionError):
run_async(func())
def test_with_6(self):
class CM:
def __aenter__(self):
return 123
def __aexit__(self, *e):
return 456
async def foo():
async with CM():
pass
with self.assertRaisesRegex(
TypeError,
"'async with' received an object from __aenter__ "
"that does not implement __await__: int"):
# it's important that __aexit__ wasn't called
run_async(foo())
def test_with_7(self):
class CM:
async def __aenter__(self):
return self
def __aexit__(self, *e):
return 444
# Exit with exception
async def foo():
async with CM():
1/0
try:
run_async(foo())
except TypeError as exc:
self.assertRegex(
exc.args[0],
"'async with' received an object from __aexit__ "
"that does not implement __await__: int")
self.assertTrue(exc.__context__ is not None)
self.assertTrue(isinstance(exc.__context__, ZeroDivisionError))
else:
self.fail('invalid asynchronous context manager did not fail')
def test_with_8(self):
CNT = 0
class CM:
async def __aenter__(self):
return self
def __aexit__(self, *e):
return 456
# Normal exit
async def foo():
nonlocal CNT
async with CM():
CNT += 1
with self.assertRaisesRegex(
TypeError,
"'async with' received an object from __aexit__ "
"that does not implement __await__: int"):
run_async(foo())
self.assertEqual(CNT, 1)
# Exit with 'break'
async def foo():
nonlocal CNT
for i in range(2):
async with CM():
CNT += 1
break
with self.assertRaisesRegex(
TypeError,
"'async with' received an object from __aexit__ "
"that does not implement __await__: int"):
run_async(foo())
self.assertEqual(CNT, 2)
# Exit with 'continue'
async def foo():
nonlocal CNT
for i in range(2):
async with CM():
CNT += 1
continue
with self.assertRaisesRegex(
TypeError,
"'async with' received an object from __aexit__ "
"that does not implement __await__: int"):
run_async(foo())
self.assertEqual(CNT, 3)
# Exit with 'return'
async def foo():
nonlocal CNT
async with CM():
CNT += 1
return
with self.assertRaisesRegex(
TypeError,
"'async with' received an object from __aexit__ "
"that does not implement __await__: int"):
run_async(foo())
self.assertEqual(CNT, 4)
def test_with_9(self):
CNT = 0
class CM:
async def __aenter__(self):
return self
async def __aexit__(self, *e):
1/0
async def foo():
nonlocal CNT
async with CM():
CNT += 1
with self.assertRaises(ZeroDivisionError):
run_async(foo())
self.assertEqual(CNT, 1)
def test_with_10(self):
CNT = 0
class CM:
async def __aenter__(self):
return self
async def __aexit__(self, *e):
1/0
async def foo():
nonlocal CNT
async with CM():
async with CM():
raise RuntimeError
try:
run_async(foo())
except ZeroDivisionError as exc:
self.assertTrue(exc.__context__ is not None)
self.assertTrue(isinstance(exc.__context__, ZeroDivisionError))
self.assertTrue(isinstance(exc.__context__.__context__,
RuntimeError))
else:
self.fail('exception from __aexit__ did not propagate')
def test_with_11(self):
CNT = 0
class CM:
async def __aenter__(self):
raise NotImplementedError
async def __aexit__(self, *e):
1/0
async def foo():
nonlocal CNT
async with CM():
raise RuntimeError
try:
run_async(foo())
except NotImplementedError as exc:
self.assertTrue(exc.__context__ is None)
else:
self.fail('exception from __aenter__ did not propagate')
def test_with_12(self):
CNT = 0
class CM:
async def __aenter__(self):
return self
async def __aexit__(self, *e):
return True
async def foo():
nonlocal CNT
async with CM() as cm:
self.assertIs(cm.__class__, CM)
raise RuntimeError
run_async(foo())
def test_with_13(self):
CNT = 0
class CM:
async def __aenter__(self):
1/0
async def __aexit__(self, *e):
return True
async def foo():
nonlocal CNT
CNT += 1
async with CM():
CNT += 1000
CNT += 10000
with self.assertRaises(ZeroDivisionError):
run_async(foo())
self.assertEqual(CNT, 1)
def test_for_1(self):
aiter_calls = 0
class AsyncIter:
def __init__(self):
self.i = 0
def __aiter__(self):
nonlocal aiter_calls
aiter_calls += 1
return self
async def __anext__(self):
self.i += 1
if not (self.i % 10):
await AsyncYield(self.i * 10)
if self.i > 100:
raise StopAsyncIteration
return self.i, self.i
buffer = []
async def test1():
async for i1, i2 in AsyncIter():
buffer.append(i1 + i2)
yielded, _ = run_async(test1())
# Make sure that __aiter__ was called only once
self.assertEqual(aiter_calls, 1)
self.assertEqual(yielded, [i * 100 for i in range(1, 11)])
self.assertEqual(buffer, [i*2 for i in range(1, 101)])
buffer = []
async def test2():
nonlocal buffer
async for i in AsyncIter():
buffer.append(i[0])
if i[0] == 20:
break
else:
buffer.append('what?')
buffer.append('end')
yielded, _ = run_async(test2())
# Make sure that __aiter__ was called only once
self.assertEqual(aiter_calls, 2)
self.assertEqual(yielded, [100, 200])
self.assertEqual(buffer, [i for i in range(1, 21)] + ['end'])
buffer = []
async def test3():
nonlocal buffer
async for i in AsyncIter():
if i[0] > 20:
continue
buffer.append(i[0])
else:
buffer.append('what?')
buffer.append('end')
yielded, _ = run_async(test3())
# Make sure that __aiter__ was called only once
self.assertEqual(aiter_calls, 3)
self.assertEqual(yielded, [i * 100 for i in range(1, 11)])
self.assertEqual(buffer, [i for i in range(1, 21)] +
['what?', 'end'])
def test_for_2(self):
tup = (1, 2, 3)
refs_before = sys.getrefcount(tup)
async def foo():
async for i in tup:
print('never going to happen')
with self.assertRaisesRegex(
TypeError, "async for' requires an object.*__aiter__.*tuple"):
run_async(foo())
self.assertEqual(sys.getrefcount(tup), refs_before)
def test_for_3(self):
class I:
def __aiter__(self):
return self
aiter = I()
refs_before = sys.getrefcount(aiter)
async def foo():
async for i in aiter:
print('never going to happen')
with self.assertRaisesRegex(
TypeError,
r"that does not implement __anext__"):
run_async(foo())
self.assertEqual(sys.getrefcount(aiter), refs_before)
def test_for_4(self):
class I:
def __aiter__(self):
return self
def __anext__(self):
return ()
aiter = I()
refs_before = sys.getrefcount(aiter)
async def foo():
async for i in aiter:
print('never going to happen')
with self.assertRaisesRegex(
TypeError,
"async for' received an invalid object.*__anext__.*tuple"):
run_async(foo())
self.assertEqual(sys.getrefcount(aiter), refs_before)
def test_for_6(self):
I = 0
class Manager:
async def __aenter__(self):
nonlocal I
I += 10000
async def __aexit__(self, *args):
nonlocal I
I += 100000
class Iterable:
def __init__(self):
self.i = 0
def __aiter__(self):
return self
async def __anext__(self):
if self.i > 10:
raise StopAsyncIteration
self.i += 1
return self.i
##############
manager = Manager()
iterable = Iterable()
mrefs_before = sys.getrefcount(manager)
irefs_before = sys.getrefcount(iterable)
async def main():
nonlocal I
async with manager:
async for i in iterable:
I += 1
I += 1000
with warnings.catch_warnings():
warnings.simplefilter("error")
# Test that __aiter__ that returns an asynchronous iterator
# directly does not throw any warnings.
run_async(main())
self.assertEqual(I, 111011)
self.assertEqual(sys.getrefcount(manager), mrefs_before)
self.assertEqual(sys.getrefcount(iterable), irefs_before)
##############
async def main():
nonlocal I
async with Manager():
async for i in Iterable():
I += 1
I += 1000
async with Manager():
async for i in Iterable():
I += 1
I += 1000
run_async(main())
self.assertEqual(I, 333033)
##############
async def main():
nonlocal I
async with Manager():
I += 100
async for i in Iterable():
I += 1
else:
I += 10000000
I += 1000
async with Manager():
I += 100
async for i in Iterable():
I += 1
else:
I += 10000000
I += 1000
run_async(main())
self.assertEqual(I, 20555255)
def test_for_7(self):
CNT = 0
class AI:
def __aiter__(self):
1/0
async def foo():
nonlocal CNT
async for i in AI():
CNT += 1
CNT += 10
with self.assertRaises(ZeroDivisionError):
run_async(foo())
self.assertEqual(CNT, 0)
def test_for_8(self):
CNT = 0
class AI:
def __aiter__(self):
1/0
async def foo():
nonlocal CNT
async for i in AI():
CNT += 1
CNT += 10
with self.assertRaises(ZeroDivisionError):
with warnings.catch_warnings():
warnings.simplefilter("error")
# Test that if __aiter__ raises an exception it propagates
# without any kind of warning.
run_async(foo())
self.assertEqual(CNT, 0)
def test_for_11(self):
class F:
def __aiter__(self):
return self
def __anext__(self):
return self
def __await__(self):
1 / 0
async def main():
async for _ in F():
pass
with self.assertRaisesRegex(TypeError,
'an invalid object from __anext__') as c:
main().send(None)
err = c.exception
self.assertIsInstance(err.__cause__, ZeroDivisionError)
def test_for_tuple(self):
class Done(Exception): pass
class AIter(tuple):
i = 0
def __aiter__(self):
return self
async def __anext__(self):
if self.i >= len(self):
raise StopAsyncIteration
self.i += 1
return self[self.i - 1]
result = []
async def foo():
async for i in AIter([42]):
result.append(i)
raise Done
with self.assertRaises(Done):
foo().send(None)
self.assertEqual(result, [42])
def test_for_stop_iteration(self):
class Done(Exception): pass
class AIter(StopIteration):
i = 0
def __aiter__(self):
return self
async def __anext__(self):
if self.i:
raise StopAsyncIteration
self.i += 1
return self.value
result = []
async def foo():
async for i in AIter(42):
result.append(i)
raise Done
with self.assertRaises(Done):
foo().send(None)
self.assertEqual(result, [42])
def test_comp_1(self):
async def f(i):
return i
async def run_list():
return [await c for c in [f(1), f(41)]]
async def run_set():
return {await c for c in [f(1), f(41)]}
async def run_dict1():
return {await c: 'a' for c in [f(1), f(41)]}
async def run_dict2():
return {i: await c for i, c in enumerate([f(1), f(41)])}
self.assertEqual(run_async(run_list()), ([], [1, 41]))
self.assertEqual(run_async(run_set()), ([], {1, 41}))
self.assertEqual(run_async(run_dict1()), ([], {1: 'a', 41: 'a'}))
self.assertEqual(run_async(run_dict2()), ([], {0: 1, 1: 41}))
def test_comp_2(self):
async def f(i):
return i
async def run_list():
return [s for c in [f(''), f('abc'), f(''), f(['de', 'fg'])]
for s in await c]
self.assertEqual(
run_async(run_list()),
([], ['a', 'b', 'c', 'de', 'fg']))
async def run_set():
return {d
for c in [f([f([10, 30]),
f([20])])]
for s in await c
for d in await s}
self.assertEqual(
run_async(run_set()),
([], {10, 20, 30}))
async def run_set2():
return {await s
for c in [f([f(10), f(20)])]
for s in await c}
self.assertEqual(
run_async(run_set2()),
([], {10, 20}))
def test_comp_3(self):
async def f(it):
for i in it:
yield i
async def run_list():
return [i + 1 async for i in f([10, 20])]
self.assertEqual(
run_async(run_list()),
([], [11, 21]))
async def run_set():
return {i + 1 async for i in f([10, 20])}
self.assertEqual(
run_async(run_set()),
([], {11, 21}))
async def run_dict():
return {i + 1: i + 2 async for i in f([10, 20])}
self.assertEqual(
run_async(run_dict()),
([], {11: 12, 21: 22}))
async def run_gen():
gen = (i + 1 async for i in f([10, 20]))
return [g + 100 async for g in gen]
self.assertEqual(
run_async(run_gen()),
([], [111, 121]))
def test_comp_4(self):
async def f(it):
for i in it:
yield i
async def run_list():
return [i + 1 async for i in f([10, 20]) if i > 10]
self.assertEqual(
run_async(run_list()),
([], [21]))
async def run_set():
return {i + 1 async for i in f([10, 20]) if i > 10}
self.assertEqual(
run_async(run_set()),
([], {21}))
async def run_dict():
return {i + 1: i + 2 async for i in f([10, 20]) if i > 10}
self.assertEqual(
run_async(run_dict()),
([], {21: 22}))
async def run_gen():
gen = (i + 1 async for i in f([10, 20]) if i > 10)
return [g + 100 async for g in gen]
self.assertEqual(
run_async(run_gen()),
([], [121]))
def test_comp_4_2(self):
async def f(it):
for i in it:
yield i
async def run_list():
return [i + 10 async for i in f(range(5)) if 0 < i < 4]
self.assertEqual(
run_async(run_list()),
([], [11, 12, 13]))
async def run_set():
return {i + 10 async for i in f(range(5)) if 0 < i < 4}
self.assertEqual(
run_async(run_set()),
([], {11, 12, 13}))
async def run_dict():
return {i + 10: i + 100 async for i in f(range(5)) if 0 < i < 4}
self.assertEqual(
run_async(run_dict()),
([], {11: 101, 12: 102, 13: 103}))
async def run_gen():
gen = (i + 10 async for i in f(range(5)) if 0 < i < 4)
return [g + 100 async for g in gen]
self.assertEqual(
run_async(run_gen()),
([], [111, 112, 113]))
def test_comp_5(self):
async def f(it):
for i in it:
yield i
async def run_list():
return [i + 1 for pair in ([10, 20], [30, 40]) if pair[0] > 10
async for i in f(pair) if i > 30]
self.assertEqual(
run_async(run_list()),
([], [41]))
def test_comp_6(self):
async def f(it):
for i in it:
yield i
async def run_list():
return [i + 1 async for seq in f([(10, 20), (30,)])
for i in seq]
self.assertEqual(
run_async(run_list()),
([], [11, 21, 31]))
def test_comp_7(self):
async def f():
yield 1
yield 2
raise Exception('aaa')
async def run_list():
return [i async for i in f()]
with self.assertRaisesRegex(Exception, 'aaa'):
run_async(run_list())
def test_comp_8(self):
async def f():
return [i for i in [1, 2, 3]]
self.assertEqual(
run_async(f()),
([], [1, 2, 3]))
def test_comp_9(self):
async def gen():
yield 1
yield 2
async def f():
l = [i async for i in gen()]
return [i for i in l]
self.assertEqual(
run_async(f()),
([], [1, 2]))
def test_comp_10(self):
async def f():
xx = {i for i in [1, 2, 3]}
return {x: x for x in xx}
self.assertEqual(
run_async(f()),
([], {1: 1, 2: 2, 3: 3}))
def test_copy(self):
async def func(): pass
coro = func()
with self.assertRaises(TypeError):
copy.copy(coro)
aw = coro.__await__()
try:
with self.assertRaises(TypeError):
copy.copy(aw)
finally:
aw.close()
def test_pickle(self):
async def func(): pass
coro = func()
for proto in range(pickle.HIGHEST_PROTOCOL + 1):
with self.assertRaises((TypeError, pickle.PicklingError)):
pickle.dumps(coro, proto)
aw = coro.__await__()
try:
for proto in range(pickle.HIGHEST_PROTOCOL + 1):
with self.assertRaises((TypeError, pickle.PicklingError)):
pickle.dumps(aw, proto)
finally:
aw.close()
def test_fatal_coro_warning(self):
# Issue 27811
async def func(): pass
with warnings.catch_warnings(), support.captured_stderr() as stderr:
warnings.filterwarnings("error")
func()
support.gc_collect()
self.assertIn("was never awaited", stderr.getvalue())
class CoroAsyncIOCompatTest(unittest.TestCase):
def test_asyncio_1(self):
# asyncio cannot be imported when Python is compiled without thread
# support
asyncio = support.import_module('asyncio')
class MyException(Exception):
pass
buffer = []
class CM:
async def __aenter__(self):
buffer.append(1)
await asyncio.sleep(0.01)
buffer.append(2)
return self
async def __aexit__(self, exc_type, exc_val, exc_tb):
await asyncio.sleep(0.01)
buffer.append(exc_type.__name__)
async def f():
async with CM() as c:
await asyncio.sleep(0.01)
raise MyException
buffer.append('unreachable')
loop = asyncio.new_event_loop()
asyncio.set_event_loop(loop)
try:
loop.run_until_complete(f())
except MyException:
pass
finally:
loop.close()
asyncio.set_event_loop(None)
self.assertEqual(buffer, [1, 2, 'MyException'])
class SysSetCoroWrapperTest(unittest.TestCase):
def test_set_wrapper_1(self):
async def foo():
return 'spam'
wrapped = None
def wrap(gen):
nonlocal wrapped
wrapped = gen
return gen
with self.assertWarns(DeprecationWarning):
self.assertIsNone(sys.get_coroutine_wrapper())
with self.assertWarns(DeprecationWarning):
sys.set_coroutine_wrapper(wrap)
with self.assertWarns(DeprecationWarning):
self.assertIs(sys.get_coroutine_wrapper(), wrap)
try:
f = foo()
self.assertTrue(wrapped)
self.assertEqual(run_async(f), ([], 'spam'))
finally:
with self.assertWarns(DeprecationWarning):
sys.set_coroutine_wrapper(None)
f.close()
with self.assertWarns(DeprecationWarning):
self.assertIsNone(sys.get_coroutine_wrapper())
wrapped = None
coro = foo()
self.assertFalse(wrapped)
coro.close()
def test_set_wrapper_2(self):
with self.assertWarns(DeprecationWarning):
self.assertIsNone(sys.get_coroutine_wrapper())
with self.assertRaisesRegex(TypeError, "callable expected, got int"):
with self.assertWarns(DeprecationWarning):
sys.set_coroutine_wrapper(1)
with self.assertWarns(DeprecationWarning):
self.assertIsNone(sys.get_coroutine_wrapper())
def test_set_wrapper_3(self):
async def foo():
return 'spam'
def wrapper(coro):
async def wrap(coro):
return await coro
return wrap(coro)
with self.assertWarns(DeprecationWarning):
sys.set_coroutine_wrapper(wrapper)
try:
with silence_coro_gc(), self.assertRaisesRegex(
RuntimeError,
r"coroutine wrapper.*\.wrapper at 0x.*attempted to "
r"recursively wrap .* wrap .*"):
foo()
finally:
with self.assertWarns(DeprecationWarning):
sys.set_coroutine_wrapper(None)
def test_set_wrapper_4(self):
@types.coroutine
def foo():
return 'spam'
wrapped = None
def wrap(gen):
nonlocal wrapped
wrapped = gen
return gen
with self.assertWarns(DeprecationWarning):
sys.set_coroutine_wrapper(wrap)
try:
foo()
self.assertIs(
wrapped, None,
"generator-based coroutine was wrapped via "
"sys.set_coroutine_wrapper")
finally:
with self.assertWarns(DeprecationWarning):
sys.set_coroutine_wrapper(None)
class OriginTrackingTest(unittest.TestCase):
def here(self):
info = inspect.getframeinfo(inspect.currentframe().f_back)
return (info.filename, info.lineno)
def test_origin_tracking(self):
orig_depth = sys.get_coroutine_origin_tracking_depth()
try:
async def corofn():
pass
sys.set_coroutine_origin_tracking_depth(0)
self.assertEqual(sys.get_coroutine_origin_tracking_depth(), 0)
with contextlib.closing(corofn()) as coro:
self.assertIsNone(coro.cr_origin)
sys.set_coroutine_origin_tracking_depth(1)
self.assertEqual(sys.get_coroutine_origin_tracking_depth(), 1)
fname, lineno = self.here()
with contextlib.closing(corofn()) as coro:
self.assertEqual(coro.cr_origin,
((fname, lineno + 1, "test_origin_tracking"),))
sys.set_coroutine_origin_tracking_depth(2)
self.assertEqual(sys.get_coroutine_origin_tracking_depth(), 2)
def nested():
return (self.here(), corofn())
fname, lineno = self.here()
((nested_fname, nested_lineno), coro) = nested()
with contextlib.closing(coro):
self.assertEqual(coro.cr_origin,
((nested_fname, nested_lineno, "nested"),
(fname, lineno + 1, "test_origin_tracking")))
# Check we handle running out of frames correctly
sys.set_coroutine_origin_tracking_depth(1000)
with contextlib.closing(corofn()) as coro:
self.assertTrue(2 < len(coro.cr_origin) < 1000)
# We can't set depth negative
with self.assertRaises(ValueError):
sys.set_coroutine_origin_tracking_depth(-1)
# And trying leaves it unchanged
self.assertEqual(sys.get_coroutine_origin_tracking_depth(), 1000)
finally:
sys.set_coroutine_origin_tracking_depth(orig_depth)
def test_origin_tracking_warning(self):
async def corofn():
pass
a1_filename, a1_lineno = self.here()
def a1():
return corofn() # comment in a1
a1_lineno += 2
a2_filename, a2_lineno = self.here()
def a2():
return a1() # comment in a2
a2_lineno += 2
def check(depth, msg):
sys.set_coroutine_origin_tracking_depth(depth)
with self.assertWarns(RuntimeWarning) as cm:
a2()
support.gc_collect()
self.assertEqual(msg, str(cm.warning))
orig_depth = sys.get_coroutine_origin_tracking_depth()
try:
msg = check(0, f"coroutine '{corofn.__qualname__}' was never awaited")
check(1, "".join([
f"coroutine '{corofn.__qualname__}' was never awaited\n",
"Coroutine created at (most recent call last)\n",
f' File "{a1_filename}", line {a1_lineno}, in a1\n',
f' return corofn() # comment in a1',
]))
check(2, "".join([
f"coroutine '{corofn.__qualname__}' was never awaited\n",
"Coroutine created at (most recent call last)\n",
f' File "{a2_filename}", line {a2_lineno}, in a2\n',
f' return a1() # comment in a2\n',
f' File "{a1_filename}", line {a1_lineno}, in a1\n',
f' return corofn() # comment in a1',
]))
finally:
sys.set_coroutine_origin_tracking_depth(orig_depth)
def test_unawaited_warning_when_module_broken(self):
# Make sure we don't blow up too bad if
# warnings._warn_unawaited_coroutine is broken somehow (e.g. because
# of shutdown problems)
async def corofn():
pass
orig_wuc = warnings._warn_unawaited_coroutine
try:
warnings._warn_unawaited_coroutine = lambda coro: 1/0
with support.captured_stderr() as stream:
corofn()
support.gc_collect()
self.assertIn("Exception ignored in", stream.getvalue())
self.assertIn("ZeroDivisionError", stream.getvalue())
self.assertIn("was never awaited", stream.getvalue())
del warnings._warn_unawaited_coroutine
with support.captured_stderr() as stream:
corofn()
support.gc_collect()
self.assertIn("was never awaited", stream.getvalue())
finally:
warnings._warn_unawaited_coroutine = orig_wuc
class UnawaitedWarningDuringShutdownTest(unittest.TestCase):
# https://bugs.python.org/issue32591#msg310726
def test_unawaited_warning_during_shutdown(self):
code = ("import asyncio\n"
"async def f(): pass\n"
"asyncio.gather(f())\n")
assert_python_ok("-c", code)
code = ("import sys\n"
"async def f(): pass\n"
"sys.coro = f()\n")
assert_python_ok("-c", code)
code = ("import sys\n"
"async def f(): pass\n"
"sys.corocycle = [f()]\n"
"sys.corocycle.append(sys.corocycle)\n")
assert_python_ok("-c", code)
@support.cpython_only
class CAPITest(unittest.TestCase):
def test_tp_await_1(self):
from _testcapi import awaitType as at
async def foo():
future = at(iter([1]))
return (await future)
self.assertEqual(foo().send(None), 1)
def test_tp_await_2(self):
# Test tp_await to __await__ mapping
from _testcapi import awaitType as at
future = at(iter([1]))
self.assertEqual(next(future.__await__()), 1)
def test_tp_await_3(self):
from _testcapi import awaitType as at
async def foo():
future = at(1)
return (await future)
with self.assertRaisesRegex(
TypeError, "__await__.*returned non-iterator of type 'int'"):
self.assertEqual(foo().send(None), 1)
if __name__=="__main__":
unittest.main()
| FFMG/myoddweb.piger | monitor/api/python/Python-3.7.2/Lib/test/test_coroutines.py | Python | gpl-2.0 | 63,767 |
#
# Copyright 2002-2006 Zuza Software Foundation
#
# This file is part of translate.
#
# translate is free software; you can redistribute it and/or modify
# it under the terms of the GNU General Public License as published by
# the Free Software Foundation; either version 2 of the License, or
# (at your option) any later version.
#
# translate is distributed in the hope that it will be useful,
# but WITHOUT ANY WARRANTY; without even the implied warranty of
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
# GNU General Public License for more details.
#
# You should have received a copy of the GNU General Public License
# along with this program; if not, see <http://www.gnu.org/licenses/>.
#
from translate.storage.xml_extract import unit_tree
# _split_xpath_component
def test__split_xpath_component():
assert ("some-tag", 0) == unit_tree._split_xpath_component("some-tag[0]")
# _split_xpath
def test__split_xpath():
assert [
("p", 4),
("text", 3),
("body", 2),
("document-content", 1),
] == unit_tree._split_xpath("document-content[1]/body[2]/text[3]/p[4]")
# _add_unit_to_tree
def make_tree_1(unit):
root = unit_tree.XPathTree()
node = root
node.children["document-content", 1] = unit_tree.XPathTree()
node = node.children["document-content", 1]
node.children["body", 1] = unit_tree.XPathTree()
node = node.children["body", 1]
node.children["text", 1] = unit_tree.XPathTree()
node = node.children["text", 1]
node.children["p", 1] = unit_tree.XPathTree()
node = node.children["p", 1]
node.unit = unit
return root
def make_tree_2(unit_1, unit_2):
root = make_tree_1(unit_1)
node = root.children["document-content", 1]
node.children["body", 2] = unit_tree.XPathTree()
node = node.children["body", 2]
node.children["text", 3] = unit_tree.XPathTree()
node = node.children["text", 3]
node.children["p", 4] = unit_tree.XPathTree()
node = node.children["p", 4]
node.unit = unit_2
return root
def test__add_unit_to_tree():
from translate.storage import xliff
xliff_file = xliff.xlifffile
# xliff_file = factory.classes['xlf']()
# Add the first unit
unit_1 = xliff_file.UnitClass("Hello")
xpath_1 = "document-content[1]/body[1]/text[1]/p[1]"
constructed_tree_1 = unit_tree.XPathTree()
unit_tree._add_unit_to_tree(
constructed_tree_1, unit_tree._split_xpath(xpath_1), unit_1
)
test_tree_1 = make_tree_1(unit_1)
assert test_tree_1 == constructed_tree_1
# Add another unit
unit_2 = xliff_file.UnitClass("World")
xpath_2 = "document-content[1]/body[2]/text[3]/p[4]"
constructed_tree_2 = make_tree_1(unit_1)
unit_tree._add_unit_to_tree(
constructed_tree_2, unit_tree._split_xpath(xpath_2), unit_2
)
test_tree_2 = make_tree_2(unit_1, unit_2)
assert test_tree_2 == constructed_tree_2
| nijel/translate | translate/storage/xml_extract/test_unit_tree.py | Python | gpl-2.0 | 2,956 |
"""JSL schema for Digester worker results."""
import jsl
from f8a_worker.schemas import JSLSchemaBaseWithRelease
# Describe v1-0-0
ROLE_v1_0_0 = "v1-0-0"
ROLE_TITLE = jsl.roles.Var({
ROLE_v1_0_0: "Digests v1-0-0"
})
class DigesterDetail(jsl.Document):
"""JSL schema for Digester worker results details."""
class Options(object):
"""JSL schema for Digester worker results details."""
definition_id = "digester_details"
description = "Details of Digester run on one file"
artifact = jsl.BooleanField() # not required
path = jsl.StringField(required=True)
ssdeep = jsl.StringField(required=True)
md5 = jsl.StringField(required=True)
sha1 = jsl.StringField(required=True)
sha256 = jsl.StringField(required=True)
class DigesterResult(JSLSchemaBaseWithRelease):
"""JSL schema for Digester worker results."""
class Options(object):
"""JSL schema for Digester worker results."""
definition_id = "digests"
description = "Result of Digester worker"
status = jsl.StringField(enum=["success", "error"], required=True)
details = jsl.ArrayField(
jsl.DocumentField(DigesterDetail, as_ref=True),
required=True
)
summary = jsl.ArrayField(jsl.StringField(), required=True)
THE_SCHEMA = DigesterResult
| jpopelka/fabric8-analytics-worker | f8a_worker/workers/schemas/digests.py | Python | gpl-3.0 | 1,331 |
from rest_framework import permissions, authentication, generics
from nodeshot.core.base.mixins import ACLMixin
from .serializers import *
from .models import *
class ServiceList(ACLMixin, generics.ListCreateAPIView):
"""
Retrieve service list according to user access level
Parameters:
* `limit=<n>`: specify number of items per page (defaults to 40)
* `limit=0`: turns off pagination
"""
authentication_classes = (authentication.SessionAuthentication,)
permission_classes = (permissions.DjangoModelPermissionsOrAnonReadOnly,)
model = Service
queryset = Service.objects.all()
serializer_class = ServiceListSerializer
pagination_serializer_class = PaginatedServiceSerializer
paginate_by_param = 'limit'
paginate_by = 40
service_list = ServiceList.as_view()
class ServiceDetail(ACLMixin, generics.RetrieveUpdateDestroyAPIView):
"""
Retrieve service detail according to user access level
"""
authentication_classes = (authentication.SessionAuthentication,)
permission_classes = (permissions.DjangoModelPermissionsOrAnonReadOnly,)
model = Service
queryset = Service.objects.all()
serializer_class = ServiceDetailSerializer
service_details = ServiceDetail.as_view()
class CategoryList(generics.ListCreateAPIView):
"""
List of service categories
"""
authentication_classes = (authentication.SessionAuthentication,)
permission_classes = (permissions.DjangoModelPermissionsOrAnonReadOnly,)
model = Category
serializer_class = CategorySerializer
service_category_list = CategoryList.as_view()
class CategoryDetail(generics.RetrieveUpdateAPIView):
"""
Category detail view
"""
authentication_classes = (authentication.SessionAuthentication,)
permission_classes = (permissions.DjangoModelPermissionsOrAnonReadOnly,)
model = Category
serializer_class = CategorySerializer
service_category_details = CategoryDetail.as_view()
| sephiroth6/nodeshot | nodeshot/networking/services/views.py | Python | gpl-3.0 | 1,999 |
from collections import namedtuple
import time
import struct
from datetime import datetime
from decocare import lib
from .. exceptions import InvalidPacketReceived
_Packet = namedtuple('Packet', [
'type', 'serial', 'op', 'payload', 'crc',
'date', 'dateString', 'valid', 'chan',
'payload_hex'
])
class Packet (_Packet):
@classmethod
def fromCommand (klass, command, payload=bytearray([0x00]), serial=None,
stamp=None, timezone=None, chan=None,
crc=None, valid=None):
stamp = stamp or time.time( )
dt = datetime.fromtimestamp(stamp).replace(tzinfo=timezone)
rftype = 0xA7
record = dict(date=stamp * 1000
, dateString=dt.isoformat( )
, type = rftype
, serial=serial
, op = command.code
, payload = payload
, payload_hex = str(payload).encode('hex')
, crc = crc
, valid = valid
, chan = chan
# , rfpacket=str(rfpacket).encode('hex')
# , head=str(head).encode('hex')
# , op=str(rfpacket[0:1]).encode('hex')
# , decocare_hex=msg
)
pkt = klass(**record)
return pkt
def update (self, payload=None, chan=None):
pkt = self
if payload:
pkt = pkt._replace(payload=payload)
pkt = pkt._replace(crc=pkt.genCRC( ))
# self.crc =
return pkt
def assemble (self):
pkt = self.update( )
buf = bytearray([self.type])
buf.extend(pkt.serial.decode('hex'))
buf.extend(bytearray([pkt.op]))
buf.extend(pkt.payload)
buf.extend(bytearray([pkt.crc]))
return buf
def oneliner (self):
kwds = dict(head=str(bytearray([self.op])).encode('hex')
, serial=self.serial
, tail=str(self.payload + bytearray([self.crc])).encode('hex')
)
return """{head}{serial}{tail}""".format(**kwds)
def genCRC (self):
buf = bytearray([self.type])
buf.extend(self.serial.decode('hex'))
buf.extend(bytearray([self.op]))
buf.extend(self.payload)
calculated = lib.CRC8.compute(buf)
return calculated
@classmethod
def fromBuffer (klass, buf, stamp=None, timezone=None, chan=None):
stamp = stamp or time.time( )
# dt = datetime.fromtimestamp(stamp).replace(tzinfo=self.args.timezone)
dt = datetime.fromtimestamp(stamp).replace(tzinfo=timezone)
msg = lib.hexdump(buf)
# head = buf[0:2]
rfpacket = buf
rftype = buf[0]
serial = str(rfpacket[1:4]).encode('hex')
command = None
payload = None
crc = None
valid = False
if serial and len(rfpacket) > 5:
rftype = rfpacket[0]
command = rfpacket[4]
payload = rfpacket[5:-1]
crc = int(rfpacket[-1])
calculated = lib.CRC8.compute(rfpacket[:-1])
valid = calculated == crc
if not valid:
raise InvalidPacketReceived
record = dict(date=stamp * 1000
, dateString=dt.isoformat( )
, type = rftype
, serial = serial
, op = command
, payload = payload
, payload_hex = str(payload).encode('hex')
, crc = crc
, valid = valid
, chan = chan
# , rfpacket=str(rfpacket).encode('hex')
# , head=str(head).encode('hex')
# , op=str(rfpacket[0:1]).encode('hex')
# , decocare_hex=msg
)
pkt = klass(**record)
return pkt
| oskarpearson/mmblelink | mmeowlink/packets/rf.py | Python | gpl-3.0 | 3,435 |
#!/usr/bin/env python2
# vim:fileencoding=UTF-8:ts=4:sw=4:sta:et:sts=4:ai
from __future__ import with_statement
__license__ = 'GPL v3'
__copyright__ = '2009, Kovid Goyal <[email protected]>'
__docformat__ = 'restructuredtext en'
import re, time
from functools import partial
from PyQt5.Qt import (
QComboBox, Qt, QLineEdit, pyqtSlot, QDialog,
pyqtSignal, QCompleter, QAction, QKeySequence, QTimer,
QIcon, QMenu, QApplication, QKeyEvent)
from calibre.gui2 import config, error_dialog, question_dialog, gprefs
from calibre.gui2.dialogs.confirm_delete import confirm
from calibre.gui2.dialogs.saved_search_editor import SavedSearchEditor
from calibre.gui2.dialogs.search import SearchDialog
class AsYouType(unicode):
def __new__(cls, text):
self = unicode.__new__(cls, text)
self.as_you_type = True
return self
class SearchLineEdit(QLineEdit): # {{{
key_pressed = pyqtSignal(object)
select_on_mouse_press = None
def keyPressEvent(self, event):
self.key_pressed.emit(event)
QLineEdit.keyPressEvent(self, event)
def dropEvent(self, ev):
self.parent().normalize_state()
return QLineEdit.dropEvent(self, ev)
def contextMenuEvent(self, ev):
self.parent().normalize_state()
menu = self.createStandardContextMenu()
menu.setAttribute(Qt.WA_DeleteOnClose)
for action in menu.actions():
if action.text().startswith(_('&Paste') + '\t'):
break
ac = menu.addAction(_('Paste and &search'))
ac.setEnabled(bool(QApplication.clipboard().text()))
ac.setIcon(QIcon(I('search.png')))
ac.triggered.connect(self.paste_and_search)
menu.insertAction(action, ac)
menu.exec_(ev.globalPos())
def paste_and_search(self):
self.paste()
ev = QKeyEvent(QKeyEvent.KeyPress, Qt.Key_Enter, Qt.NoModifier)
self.keyPressEvent(ev)
@pyqtSlot()
def paste(self, *args):
self.parent().normalize_state()
return QLineEdit.paste(self)
def focusInEvent(self, ev):
self.select_on_mouse_press = time.time()
return QLineEdit.focusInEvent(self, ev)
def mousePressEvent(self, ev):
QLineEdit.mousePressEvent(self, ev)
if self.select_on_mouse_press is not None and abs(time.time() - self.select_on_mouse_press) < 0.2:
self.selectAll()
self.select_on_mouse_press = None
# }}}
class SearchBox2(QComboBox): # {{{
'''
To use this class:
* Call initialize()
* Connect to the search() and cleared() signals from this widget.
* Connect to the changed() signal to know when the box content changes
* Connect to focus_to_library() signal to be told to manually change focus
* Call search_done() after every search is complete
* Call set_search_string() to perform a search programmatically
* You can use the current_text property to get the current search text
Be aware that if you are using it in a slot connected to the
changed() signal, if the connection is not queued it will not be
accurate.
'''
INTERVAL = 1500 #: Time to wait before emitting search signal
MAX_COUNT = 25
search = pyqtSignal(object)
cleared = pyqtSignal()
changed = pyqtSignal()
focus_to_library = pyqtSignal()
def __init__(self, parent=None):
QComboBox.__init__(self, parent)
self.normal_background = 'rgb(255, 255, 255, 0%)'
self.line_edit = SearchLineEdit(self)
self.setLineEdit(self.line_edit)
c = self.line_edit.completer()
c.setCompletionMode(c.PopupCompletion)
c.highlighted[str].connect(self.completer_used)
self.line_edit.key_pressed.connect(self.key_pressed, type=Qt.DirectConnection)
# QueuedConnection as workaround for https://bugreports.qt-project.org/browse/QTBUG-40807
self.activated[str].connect(self.history_selected, type=Qt.QueuedConnection)
self.setEditable(True)
self.as_you_type = True
self.timer = QTimer()
self.timer.setSingleShot(True)
self.timer.timeout.connect(self.timer_event, type=Qt.QueuedConnection)
self.setInsertPolicy(self.NoInsert)
self.setMaxCount(self.MAX_COUNT)
self.setSizeAdjustPolicy(self.AdjustToMinimumContentsLengthWithIcon)
self.setMinimumContentsLength(25)
self._in_a_search = False
self.tool_tip_text = self.toolTip()
def initialize(self, opt_name, colorize=False, help_text=_('Search')):
self.as_you_type = config['search_as_you_type']
self.opt_name = opt_name
items = []
for item in config[opt_name]:
if item not in items:
items.append(item)
self.addItems(items)
self.line_edit.setPlaceholderText(help_text)
self.colorize = colorize
self.clear()
def hide_completer_popup(self):
try:
self.lineEdit().completer().popup().setVisible(False)
except:
pass
def normalize_state(self):
self.setToolTip(self.tool_tip_text)
self.line_edit.setStyleSheet(
'QLineEdit{color:none;background-color:%s;}' % self.normal_background)
def text(self):
return self.currentText()
def clear_history(self, *args):
QComboBox.clear(self)
def clear(self, emit_search=True):
self.normalize_state()
self.setEditText('')
if emit_search:
self.search.emit('')
self._in_a_search = False
self.cleared.emit()
def clear_clicked(self, *args):
self.clear()
def search_done(self, ok):
if isinstance(ok, basestring):
self.setToolTip(ok)
ok = False
if not unicode(self.currentText()).strip():
self.clear(emit_search=False)
return
self._in_a_search = ok
col = 'rgba(0,255,0,20%)' if ok else 'rgb(255,0,0,20%)'
if not self.colorize:
col = self.normal_background
self.line_edit.setStyleSheet('QLineEdit{color:black;background-color:%s;}' % col)
# Comes from the lineEdit control
def key_pressed(self, event):
k = event.key()
if k in (Qt.Key_Left, Qt.Key_Right, Qt.Key_Up, Qt.Key_Down,
Qt.Key_Home, Qt.Key_End, Qt.Key_PageUp, Qt.Key_PageDown,
Qt.Key_unknown):
return
self.normalize_state()
if self._in_a_search:
self.changed.emit()
self._in_a_search = False
if event.key() in (Qt.Key_Return, Qt.Key_Enter):
self.do_search()
self.focus_to_library.emit()
elif self.as_you_type and unicode(event.text()):
self.timer.start(1500)
# Comes from the combobox itself
def keyPressEvent(self, event):
k = event.key()
if k in (Qt.Key_Enter, Qt.Key_Return):
return self.do_search()
if k not in (Qt.Key_Up, Qt.Key_Down):
QComboBox.keyPressEvent(self, event)
else:
self.blockSignals(True)
self.normalize_state()
QComboBox.keyPressEvent(self, event)
self.blockSignals(False)
def completer_used(self, text):
self.timer.stop()
self.normalize_state()
def timer_event(self):
self._do_search(as_you_type=True)
def history_selected(self, text):
self.changed.emit()
self.do_search()
def _do_search(self, store_in_history=True, as_you_type=False):
self.hide_completer_popup()
text = unicode(self.currentText()).strip()
if not text:
return self.clear()
if as_you_type:
text = AsYouType(text)
self.search.emit(text)
if store_in_history:
idx = self.findText(text, Qt.MatchFixedString|Qt.MatchCaseSensitive)
self.block_signals(True)
if idx < 0:
self.insertItem(0, text)
else:
t = self.itemText(idx)
self.removeItem(idx)
self.insertItem(0, t)
self.setCurrentIndex(0)
self.block_signals(False)
history = [unicode(self.itemText(i)) for i in
range(self.count())]
config[self.opt_name] = history
def do_search(self, *args):
self._do_search()
def block_signals(self, yes):
self.blockSignals(yes)
self.line_edit.blockSignals(yes)
def set_search_string(self, txt, store_in_history=False, emit_changed=True):
if not store_in_history:
self.activated[str].disconnect()
try:
self.setFocus(Qt.OtherFocusReason)
if not txt:
self.clear()
else:
self.normalize_state()
# must turn on case sensitivity here so that tag browser strings
# are not case-insensitively replaced from history
self.line_edit.completer().setCaseSensitivity(Qt.CaseSensitive)
self.setEditText(txt)
self.line_edit.end(False)
if emit_changed:
self.changed.emit()
self._do_search(store_in_history=store_in_history)
self.line_edit.completer().setCaseSensitivity(Qt.CaseInsensitive)
self.focus_to_library.emit()
finally:
if not store_in_history:
# QueuedConnection as workaround for https://bugreports.qt-project.org/browse/QTBUG-40807
self.activated[str].connect(self.history_selected, type=Qt.QueuedConnection)
def search_as_you_type(self, enabled):
self.as_you_type = enabled
def in_a_search(self):
return self._in_a_search
@property
def current_text(self):
return unicode(self.lineEdit().text())
# }}}
class SavedSearchBox(QComboBox): # {{{
'''
To use this class:
* Call initialize()
* Connect to the changed() signal from this widget
if you care about changes to the list of saved searches.
'''
changed = pyqtSignal()
def __init__(self, parent=None):
QComboBox.__init__(self, parent)
self.normal_background = 'rgb(255, 255, 255, 0%)'
self.line_edit = SearchLineEdit(self)
self.setLineEdit(self.line_edit)
self.line_edit.key_pressed.connect(self.key_pressed, type=Qt.DirectConnection)
self.activated[str].connect(self.saved_search_selected)
# Turn off auto-completion so that it doesn't interfere with typing
# names of new searches.
completer = QCompleter(self)
self.setCompleter(completer)
self.setEditable(True)
self.setInsertPolicy(self.NoInsert)
self.setSizeAdjustPolicy(self.AdjustToMinimumContentsLengthWithIcon)
self.setMinimumContentsLength(10)
self.tool_tip_text = self.toolTip()
def initialize(self, _search_box, colorize=False, help_text=_('Search')):
self.search_box = _search_box
try:
self.line_edit.setPlaceholderText(help_text)
except:
# Using Qt < 4.7
pass
self.colorize = colorize
self.clear()
def normalize_state(self):
# need this because line_edit will call it in some cases such as paste
pass
def clear(self):
QComboBox.clear(self)
self.initialize_saved_search_names()
self.setEditText('')
self.line_edit.home(False)
def key_pressed(self, event):
if event.key() in (Qt.Key_Return, Qt.Key_Enter):
self.saved_search_selected(self.currentText())
def saved_search_selected(self, qname):
from calibre.gui2.ui import get_gui
db = get_gui().current_db
qname = unicode(qname)
if qname is None or not qname.strip():
self.search_box.clear()
return
if not db.saved_search_lookup(qname):
self.search_box.clear()
self.setEditText(qname)
return
self.search_box.set_search_string(u'search:"%s"' % qname, emit_changed=False)
self.setEditText(qname)
self.setToolTip(db.saved_search_lookup(qname))
def initialize_saved_search_names(self):
from calibre.gui2.ui import get_gui
gui = get_gui()
try:
names = gui.current_db.saved_search_names()
except AttributeError:
# Happens during gui initialization
names = []
self.addItems(names)
self.setCurrentIndex(-1)
# SIGNALed from the main UI
def save_search_button_clicked(self):
from calibre.gui2.ui import get_gui
db = get_gui().current_db
name = unicode(self.currentText())
if not name.strip():
name = unicode(self.search_box.text()).replace('"', '')
name = name.replace('\\', '')
if not name:
error_dialog(self, _('Create saved search'),
_('Invalid saved search name. '
'It must contain at least one letter or number'), show=True)
return
if not self.search_box.text():
error_dialog(self, _('Create saved search'),
_('There is no search to save'), show=True)
return
db.saved_search_delete(name)
db.saved_search_add(name, unicode(self.search_box.text()))
# now go through an initialization cycle to ensure that the combobox has
# the new search in it, that it is selected, and that the search box
# references the new search instead of the text in the search.
self.clear()
self.setCurrentIndex(self.findText(name))
self.saved_search_selected(name)
self.changed.emit()
def delete_current_search(self):
from calibre.gui2.ui import get_gui
db = get_gui().current_db
idx = self.currentIndex()
if idx <= 0:
error_dialog(self, _('Delete current search'),
_('No search is selected'), show=True)
return
if not confirm('<p>'+_('The selected search will be '
'<b>permanently deleted</b>. Are you sure?') +
'</p>', 'saved_search_delete', self):
return
ss = db.saved_search_lookup(unicode(self.currentText()))
if ss is None:
return
db.saved_search_delete(unicode(self.currentText()))
self.clear()
self.search_box.clear()
self.changed.emit()
# SIGNALed from the main UI
def copy_search_button_clicked(self):
from calibre.gui2.ui import get_gui
db = get_gui().current_db
idx = self.currentIndex()
if idx < 0:
return
self.search_box.set_search_string(db.saved_search_lookup(unicode(self.currentText())))
# }}}
class SearchBoxMixin(object): # {{{
def __init__(self, *args, **kwargs):
pass
def init_search_box_mixin(self):
self.search.initialize('main_search_history', colorize=True,
help_text=_('Search (For Advanced Search click the button to the left)'))
self.search.cleared.connect(self.search_box_cleared)
# Queued so that search.current_text will be correct
self.search.changed.connect(self.search_box_changed,
type=Qt.QueuedConnection)
self.search.focus_to_library.connect(self.focus_to_library)
self.clear_button.clicked.connect(self.search.clear_clicked)
self.advanced_search_button.clicked[bool].connect(self.do_advanced_search)
self.search.clear()
self.search.setMaximumWidth(self.width()-150)
self.action_focus_search = QAction(self)
shortcuts = list(
map(lambda x:unicode(x.toString(QKeySequence.PortableText)),
QKeySequence.keyBindings(QKeySequence.Find)))
shortcuts += ['/', 'Alt+S']
self.keyboard.register_shortcut('start search', _('Start search'),
default_keys=shortcuts, action=self.action_focus_search)
self.action_focus_search.triggered.connect(self.focus_search_box)
self.addAction(self.action_focus_search)
self.search.setStatusTip(re.sub(r'<\w+>', ' ',
unicode(self.search.toolTip())))
self.advanced_search_button.setStatusTip(self.advanced_search_button.toolTip())
self.clear_button.setStatusTip(self.clear_button.toolTip())
self.set_highlight_only_button_icon()
self.highlight_only_button.clicked.connect(self.highlight_only_clicked)
tt = _('Enable or disable search highlighting.') + '<br><br>'
tt += config.help('highlight_search_matches')
self.highlight_only_button.setToolTip(tt)
self.highlight_only_action = ac = QAction(self)
self.addAction(ac), ac.triggered.connect(self.highlight_only_clicked)
self.keyboard.register_shortcut('highlight search results', _('Highlight search results'), action=self.highlight_only_action)
def highlight_only_clicked(self, state):
if not config['highlight_search_matches'] and not question_dialog(self, _('Are you sure?'),
_('This will change how searching works. When you search, instead of showing only the '
'matching books, all books will be shown with the matching books highlighted. '
'Are you sure this is what you want?'), skip_dialog_name='confirm_search_highlight_toggle'):
return
config['highlight_search_matches'] = not config['highlight_search_matches']
self.set_highlight_only_button_icon()
self.search.do_search()
self.focus_to_library()
def set_highlight_only_button_icon(self):
if config['highlight_search_matches']:
self.highlight_only_button.setIcon(QIcon(I('highlight_only_on.png')))
else:
self.highlight_only_button.setIcon(QIcon(I('highlight_only_off.png')))
self.highlight_only_button.setVisible(gprefs['show_highlight_toggle_button'])
self.library_view.model().set_highlight_only(config['highlight_search_matches'])
def focus_search_box(self, *args):
self.search.setFocus(Qt.OtherFocusReason)
self.search.lineEdit().selectAll()
def search_box_cleared(self):
self.tags_view.clear()
self.saved_search.clear()
self.set_number_of_books_shown()
def search_box_changed(self):
self.saved_search.clear()
self.tags_view.conditional_clear(self.search.current_text)
def do_advanced_search(self, *args):
d = SearchDialog(self, self.library_view.model().db)
if d.exec_() == QDialog.Accepted:
self.search.set_search_string(d.search_string(), store_in_history=True)
def do_search_button(self):
self.search.do_search()
self.focus_to_library()
def focus_to_library(self):
self.current_view().setFocus(Qt.OtherFocusReason)
# }}}
class SavedSearchBoxMixin(object): # {{{
def __init__(self, *args, **kwargs):
pass
def init_saved_seach_box_mixin(self):
self.saved_search.changed.connect(self.saved_searches_changed)
self.clear_button.clicked.connect(self.saved_search.clear)
self.save_search_button.clicked.connect(
self.saved_search.save_search_button_clicked)
self.copy_search_button.clicked.connect(
self.saved_search.copy_search_button_clicked)
# self.saved_searches_changed()
self.saved_search.initialize(self.search, colorize=True,
help_text=_('Saved Searches'))
self.saved_search.setToolTip(
_('Choose saved search or enter name for new saved search'))
self.saved_search.setStatusTip(self.saved_search.toolTip())
for x in ('copy', 'save'):
b = getattr(self, x+'_search_button')
b.setStatusTip(b.toolTip())
self.save_search_button.setToolTip('<p>' +
_("Save current search under the name shown in the box. "
"Press and hold for a pop-up options menu.") + '</p>')
self.save_search_button.setMenu(QMenu())
self.save_search_button.menu().addAction(
QIcon(I('plus.png')),
_('Create saved search'),
self.saved_search.save_search_button_clicked)
self.save_search_button.menu().addAction(
QIcon(I('trash.png')), _('Delete saved search'), self.saved_search.delete_current_search)
self.save_search_button.menu().addAction(
QIcon(I('search.png')), _('Manage saved searches'), partial(self.do_saved_search_edit, None))
def saved_searches_changed(self, set_restriction=None, recount=True):
self.build_search_restriction_list()
if recount:
self.tags_view.recount()
if set_restriction: # redo the search restriction if there was one
self.apply_named_search_restriction(set_restriction)
def do_saved_search_edit(self, search):
d = SavedSearchEditor(self, search)
d.exec_()
if d.result() == d.Accepted:
self.do_rebuild_saved_searches()
def do_rebuild_saved_searches(self):
self.saved_searches_changed()
self.saved_search.clear()
# }}}
| hazrpg/calibre | src/calibre/gui2/search_box.py | Python | gpl-3.0 | 21,498 |
# -*- coding: utf-8 -*-
#
# Copyright (C) Pootle contributors.
#
# This file is a part of the Pootle project. It is distributed under the GPL3
# or later license. See the LICENSE file for a copy of the license and the
# AUTHORS file for copyright and authorship information.
import urlparse
from django import forms
from django.contrib.auth import get_user_model
from django.utils.translation import ugettext_lazy as _
class EditUserForm(forms.ModelForm):
class Meta(object):
model = get_user_model()
fields = ('full_name', 'twitter', 'linkedin', 'website', 'bio')
def clean_linkedin(self):
url = self.cleaned_data['linkedin']
if url != '':
parsed = urlparse.urlparse(url)
if 'linkedin.com' not in parsed.netloc or parsed.path == '/':
raise forms.ValidationError(
_('Please enter a valid LinkedIn user profile URL.')
)
return url
| Finntack/pootle | pootle/apps/pootle_profile/forms.py | Python | gpl-3.0 | 960 |
# This Source Code Form is subject to the terms of the Mozilla Public
# License, v. 2.0. If a copy of the MPL was not distributed with this file,
# You can obtain one at http://mozilla.org/MPL/2.0/.
import base64
import hashlib
import json
import os
import subprocess
import tempfile
import threading
import urlparse
import uuid
from collections import defaultdict
from mozprocess import ProcessHandler
from .base import (ExecutorException,
Protocol,
RefTestImplementation,
testharness_result_converter,
reftest_result_converter)
from .process import ProcessTestExecutor
from ..browsers.base import browser_command
hosts_text = """127.0.0.1 web-platform.test
127.0.0.1 www.web-platform.test
127.0.0.1 www1.web-platform.test
127.0.0.1 www2.web-platform.test
127.0.0.1 xn--n8j6ds53lwwkrqhv28a.web-platform.test
127.0.0.1 xn--lve-6lad.web-platform.test
"""
def make_hosts_file():
hosts_fd, hosts_path = tempfile.mkstemp()
with os.fdopen(hosts_fd, "w") as f:
f.write(hosts_text)
return hosts_path
class ServoTestharnessExecutor(ProcessTestExecutor):
convert_result = testharness_result_converter
def __init__(self, browser, server_config, timeout_multiplier=1, debug_info=None,
pause_after_test=False):
ProcessTestExecutor.__init__(self, browser, server_config,
timeout_multiplier=timeout_multiplier,
debug_info=debug_info)
self.pause_after_test = pause_after_test
self.result_data = None
self.result_flag = None
self.protocol = Protocol(self, browser)
self.hosts_path = make_hosts_file()
def teardown(self):
try:
os.unlink(self.hosts_path)
except OSError:
pass
ProcessTestExecutor.teardown(self)
def do_test(self, test):
self.result_data = None
self.result_flag = threading.Event()
debug_args, command = browser_command(self.binary, ["--cpu", "--hard-fail", "-z", self.test_url(test)],
self.debug_info)
self.command = command
if self.pause_after_test:
self.command.remove("-z")
self.command = debug_args + self.command
env = os.environ.copy()
env["HOST_FILE"] = self.hosts_path
if not self.interactive:
self.proc = ProcessHandler(self.command,
processOutputLine=[self.on_output],
onFinish=self.on_finish,
env=env,
storeOutput=False)
self.proc.run()
else:
self.proc = subprocess.Popen(self.command, env=env)
try:
timeout = test.timeout * self.timeout_multiplier
# Now wait to get the output we expect, or until we reach the timeout
if not self.interactive and not self.pause_after_test:
wait_timeout = timeout + 5
self.result_flag.wait(wait_timeout)
else:
wait_timeout = None
self.proc.wait()
proc_is_running = True
if self.result_flag.is_set() and self.result_data is not None:
self.result_data["test"] = test.url
result = self.convert_result(test, self.result_data)
else:
if self.proc.poll() is not None:
result = (test.result_cls("CRASH", None), [])
proc_is_running = False
else:
result = (test.result_cls("TIMEOUT", None), [])
if proc_is_running:
if self.pause_after_test:
self.logger.info("Pausing until the browser exits")
self.proc.wait()
else:
self.proc.kill()
except KeyboardInterrupt:
self.proc.kill()
raise
return result
def on_output(self, line):
prefix = "ALERT: RESULT: "
line = line.decode("utf8", "replace")
if line.startswith(prefix):
self.result_data = json.loads(line[len(prefix):])
self.result_flag.set()
else:
if self.interactive:
print line
else:
self.logger.process_output(self.proc.pid,
line,
" ".join(self.command))
def on_finish(self):
self.result_flag.set()
class TempFilename(object):
def __init__(self, directory):
self.directory = directory
self.path = None
def __enter__(self):
self.path = os.path.join(self.directory, str(uuid.uuid4()))
return self.path
def __exit__(self, *args, **kwargs):
try:
os.unlink(self.path)
except OSError:
pass
class ServoRefTestExecutor(ProcessTestExecutor):
convert_result = reftest_result_converter
def __init__(self, browser, server_config, binary=None, timeout_multiplier=1,
screenshot_cache=None, debug_info=None, pause_after_test=False):
ProcessTestExecutor.__init__(self,
browser,
server_config,
timeout_multiplier=timeout_multiplier,
debug_info=debug_info)
self.protocol = Protocol(self, browser)
self.screenshot_cache = screenshot_cache
self.implementation = RefTestImplementation(self)
self.tempdir = tempfile.mkdtemp()
self.hosts_path = make_hosts_file()
def teardown(self):
try:
os.unlink(self.hosts_path)
except OSError:
pass
os.rmdir(self.tempdir)
ProcessTestExecutor.teardown(self)
def screenshot(self, test):
full_url = self.test_url(test)
with TempFilename(self.tempdir) as output_path:
self.command = [self.binary, "--cpu", "--hard-fail", "--exit",
"-Z", "disable-text-aa,disable-canvas-aa", "--output=%s" % output_path,
full_url]
env = os.environ.copy()
env["HOST_FILE"] = self.hosts_path
self.proc = ProcessHandler(self.command,
processOutputLine=[self.on_output],
env=env)
try:
self.proc.run()
rv = self.proc.wait(timeout=test.timeout)
except KeyboardInterrupt:
self.proc.kill()
raise
if rv is None:
self.proc.kill()
return False, ("EXTERNAL-TIMEOUT", None)
if rv != 0 or not os.path.exists(output_path):
return False, ("CRASH", None)
with open(output_path) as f:
# Might need to strip variable headers or something here
data = f.read()
return True, base64.b64encode(data)
def do_test(self, test):
result = self.implementation.run_test(test)
return self.convert_result(test, result)
def on_output(self, line):
line = line.decode("utf8", "replace")
if self.interactive:
print line
else:
self.logger.process_output(self.proc.pid,
line,
" ".join(self.command))
| GreenRecycleBin/servo | tests/wpt/harness/wptrunner/executors/executorservo.py | Python | mpl-2.0 | 7,684 |
"""
This file contains counting conditions for use for count matrix based code
clone detection. (See http://goo.gl/8UuAW5 for general information about the
algorithm.)
"""
from coalib.bearlib.parsing.clang.cindex import CursorKind
from coalib.misc.Enum import enum
def is_function_declaration(cursor):
"""
Checks if the given clang cursor is a function declaration.
:param cursor: A clang cursor from the AST.
:return: A bool.
"""
return cursor.kind == CursorKind.FUNCTION_DECL
def get_identifier_name(cursor):
"""
Retrieves the identifier name from the given clang cursor.
:param cursor: A clang cursor from the AST.
:return: The identifier as string.
"""
return cursor.displayname.decode()
def is_literal(cursor):
"""
:param cursor: A clang cursor from the AST.
:return: True if the cursor is a literal of any kind..
"""
return cursor.kind in [CursorKind.INTEGER_LITERAL,
CursorKind.FLOATING_LITERAL,
CursorKind.IMAGINARY_LITERAL,
CursorKind.STRING_LITERAL,
CursorKind.CHARACTER_LITERAL,
CursorKind.OBJC_STRING_LITERAL,
CursorKind.CXX_BOOL_LITERAL_EXPR,
CursorKind.CXX_NULL_PTR_LITERAL_EXPR]
def is_reference(cursor):
"""
Determines if the cursor is a reference to something, i.e. an identifier
of a function or variable.
:param cursor: A clang cursor from the AST.
:return: True if the cursor is a reference.
"""
return cursor.kind in [CursorKind.VAR_DECL,
CursorKind.PARM_DECL,
CursorKind.DECL_REF_EXPR]
def _stack_contains_kind(stack, kind):
"""
Checks if a cursor with the given kind is within the stack.
:param stack: The stack holding a tuple holding the parent cursors and the
child number.
:param kind: The kind of the cursor to search for.
:return: True if the kind was found.
"""
for elem, dummy in stack:
if elem.kind == kind:
return True
return False
def _is_nth_child_of_kind(stack, allowed_nums, kind):
"""
Checks if the stack contains a cursor which is of the given kind and the
stack also has a child of this element which number is in the allowed_nums
list.
:param stack: The stack holding a tuple holding the parent cursors
and the child number.
:param allowed_nums: List/iterator of child numbers allowed.
:param kind: The kind of the parent element.
:return: Number of matches.
"""
is_kind_child = False
count = 0
for elem, child_num in stack:
if is_kind_child and child_num in allowed_nums:
count += 1
if elem.kind == kind:
is_kind_child = True
else:
is_kind_child = False
return count
def is_function(stack):
"""
Checks if the cursor on top of the stack is used as a method or as a
variable.
:param stack: A stack holding a tuple holding the parent cursors and the
child number.
:return: True if this is used as a function, false otherwise.
"""
return _is_nth_child_of_kind(stack, [0], CursorKind.CALL_EXPR) != 0
FOR_POSITION = enum("UNKNOWN", "INIT", "COND", "INC", "BODY")
def _get_position_in_for_tokens(tokens, position):
"""
Retrieves the semantic position of the given position in a for loop. It
operates under the assumptions that the given tokens represent a for loop
and that the given position is within the tokens.
:param tokens: The tokens representing the for loop (clang extent)
:param position: A tuple holding (line, column) of the position to
identify.
:return: A FOR_POSITION object indicating where the position is
semantically.
"""
state = FOR_POSITION.INIT
next_state = state
opened_brackets = 0
for token in tokens:
if token.spelling.decode() == ";":
next_state = state + 1
elif token.spelling.decode() == "(":
opened_brackets += 1
elif token.spelling.decode() == ")":
opened_brackets -= 1
# Closed bracket for for condition, otherwise syntax error by clang
if opened_brackets == 0:
next_state = FOR_POSITION.BODY
if next_state is not state:
token_position = (token.extent.start.line,
token.extent.start.column)
if position <= token_position:
return state
# Last state, if we reach it the position must be in body
elif next_state == FOR_POSITION.BODY:
return next_state
state = next_state
# We probably have a macro here, clang doesn't preprocess them. I don't see
# a chance of getting macros parsed right here in the limited time
# available. For our heuristic approach we'll just not count for loops
# realized through macros. FIXME: This is not covered in the tests because
# it contains a known bug that needs to be fixed, that is: macros destroy
# everything.
return FOR_POSITION.UNKNOWN # pragma: no cover
def _get_positions_in_for_loop(stack):
"""
Investigates all FOR_STMT objects in the stack and checks for each in
what position the given cursor is.
:param cursor: The cursor to investigate.
:param stack: The stack of parental cursors.
:return: A list of semantic FOR_POSITION's within for loops.
"""
results = []
for elem, dummy in stack:
if elem.kind == CursorKind.FOR_STMT:
results.append(_get_position_in_for_tokens(
elem.get_tokens(),
(stack[-1][0].location.line, stack[-1][0].location.column)))
return results
def _get_binop_operator(cursor):
"""
Returns the operator token of a binary operator cursor.
:param cursor: A cursor of kind BINARY_OPERATOR.
:return: The token object containing the actual operator or None.
"""
children = list(cursor.get_children())
operator_min_begin = (children[0].location.line,
children[0].location.column)
operator_max_end = (children[1].location.line,
children[1].location.column)
for token in cursor.get_tokens():
if (operator_min_begin < (token.extent.start.line,
token.extent.start.column) and
operator_max_end >= (token.extent.end.line,
token.extent.end.column)):
return token
return None # pragma: no cover
def _stack_contains_operators(stack, operators):
"""
Checks if one of the given operators is within the stack.
:param stack: The stack holding a tuple holding the parent cursors
and the child number.
:param operators: A list of strings. E.g. ["+", "-"]
:return: True if the operator was found.
"""
for elem, dummy in stack:
if elem.kind in [CursorKind.BINARY_OPERATOR,
CursorKind.COMPOUND_ASSIGNMENT_OPERATOR]:
operator = _get_binop_operator(elem)
# Not known how to reproduce but may be possible when evil macros
# join the game.
if operator is None: # pragma: no cover
continue
if operator.spelling.decode() in operators:
return True
return False
ARITH_BINARY_OPERATORS = ['+', '-', '*', '/', '%', '&', '|']
COMPARISION_OPERATORS = ["==", "<=", ">=", "<", ">", "!=", "&&", "||"]
ADV_ASSIGNMENT_OPERATORS = [op + "=" for op in ARITH_BINARY_OPERATORS]
ASSIGNMENT_OPERATORS = ["="] + ADV_ASSIGNMENT_OPERATORS
def in_sum(stack):
"""
A counting condition returning true if the variable is used in a sum
statement, i.e. within the operators +, - and their associated compound
operators.
"""
return _stack_contains_operators(stack, ['+', '-', '+=', '-='])
def in_product(stack):
"""
A counting condition returning true if the variable is used in a product
statement, i.e. within the operators *, /, % and their associated compound
operators.
"""
return _stack_contains_operators(stack, ['*', '/', '%', '*=', '/=', '%='])
def in_binary_operation(stack):
"""
A counting condition returning true if the variable is used in a binary
operation, i.e. within the operators |, & and their associated compound
operators.
"""
return _stack_contains_operators(stack, ['&', '|', '&=', '|='])
def member_accessed(stack):
"""
Returns true if a member of the cursor is accessed or the cursor is the
accessed member.
"""
return _stack_contains_kind(stack, CursorKind.MEMBER_REF_EXPR)
# pylint: disabled=unused-argument
def used(stack):
"""
Returns true.
"""
return True
def returned(stack):
"""
Returns true if the cursor on top is used in a return statement.
"""
return _stack_contains_kind(stack, CursorKind.RETURN_STMT)
def is_inc_or_dec(stack):
"""
Returns true if the cursor on top is inc- or decremented.
"""
for elem, dummy in stack:
if elem.kind == CursorKind.UNARY_OPERATOR:
for token in elem.get_tokens():
if token.spelling.decode() in ["--", "++"]:
return True
return False
def is_condition(stack):
"""
Returns true if the cursor on top is used as a condition.
"""
return (_is_nth_child_of_kind(stack, [0], CursorKind.WHILE_STMT) != 0 or
_is_nth_child_of_kind(stack, [0], CursorKind.IF_STMT) != 0 or
FOR_POSITION.COND in _get_positions_in_for_loop(stack))
def in_condition(stack):
"""
Returns true if the cursor on top is in the body of one condition.
"""
# In every case the first child of IF_STMT is the condition itself
# (non-NULL) so the second and third child are in the then/else branch
return _is_nth_child_of_kind(stack, [1, 2], CursorKind.IF_STMT) == 1
def in_second_level_condition(stack):
"""
Returns true if the cursor on top is in the body of two nested conditions.
"""
return _is_nth_child_of_kind(stack, [1, 2], CursorKind.IF_STMT) == 2
def in_third_level_condition(stack):
"""
Returns true if the cursor on top is in the body of three or more nested
conditions.
"""
return _is_nth_child_of_kind(stack, [1, 2], CursorKind.IF_STMT) > 2
def is_assignee(stack):
"""
Returns true if the cursor on top is assigned something.
"""
cursor_pos = (stack[-1][0].extent.end.line, stack[-1][0].extent.end.column)
for elem, dummy in stack:
if (
elem.kind == CursorKind.BINARY_OPERATOR or
elem.kind == CursorKind.COMPOUND_ASSIGNMENT_OPERATOR):
for token in elem.get_tokens():
token_pos = (token.extent.start.line,
token.extent.start.column)
# This needs to be an assignment and cursor has to be on LHS
if (
token.spelling.decode() in ASSIGNMENT_OPERATORS and
cursor_pos <= token_pos):
return True
return is_inc_or_dec(stack)
def is_assigner(stack):
"""
Returns true if the cursor on top is used for an assignment on the RHS.
"""
cursor_pos = (stack[-1][0].extent.start.line,
stack[-1][0].extent.start.column)
for elem, dummy in stack:
if (
elem.kind == CursorKind.BINARY_OPERATOR or
elem.kind == CursorKind.COMPOUND_ASSIGNMENT_OPERATOR):
for token in elem.get_tokens():
token_pos = (token.extent.end.line, token.extent.end.column)
# This needs to be an assignment and cursor has to be on RHS
# or if we have something like += its irrelevant on which side
# it is because += reads on both sides
if (token.spelling.decode() in ASSIGNMENT_OPERATORS and (
token_pos <= cursor_pos or
token.spelling.decode() != "=")):
return True
return is_inc_or_dec(stack)
def _loop_level(stack):
"""
Investigates the stack to determine the loop level.
:param stack: A stack of clang cursors.
:return: An integer representing the level of nested loops.
"""
positions_in_for = _get_positions_in_for_loop(stack)
return (positions_in_for.count(FOR_POSITION.INC) +
positions_in_for.count(FOR_POSITION.BODY) +
_is_nth_child_of_kind(stack, [1], CursorKind.WHILE_STMT))
def loop_content(stack):
"""
Returns true if the cursor on top is within a first level loop.
"""
return _loop_level(stack) == 1
def second_level_loop_content(stack):
"""
Returns true if the cursor on top is within a second level loop.
"""
return _loop_level(stack) == 2
def third_level_loop_content(stack):
"""
Returns true if the cursor on top is within a third (or higher) level loop.
"""
return _loop_level(stack) > 2
def is_param(stack):
"""
Returns true if the cursor on top is a parameter declaration.
"""
return stack[-1][0].kind == CursorKind.PARM_DECL
def is_called(stack):
"""
Yields true if the cursor is a function that is called. (Function pointers
are counted too.)
"""
return (_stack_contains_kind(stack, CursorKind.CALL_EXPR) and
is_function(stack))
def is_call_param(stack):
"""
Yields true if the cursor is a parameter to another function.
"""
return (_stack_contains_kind(stack, CursorKind.CALL_EXPR) and
not is_function(stack))
condition_dict = {"used": used,
"returned": returned,
"is_condition": is_condition,
"in_condition": in_condition,
"in_second_level_condition": in_second_level_condition,
"in_third_level_condition": in_third_level_condition,
"is_assignee": is_assignee,
"is_assigner": is_assigner,
"loop_content": loop_content,
"second_level_loop_content": second_level_loop_content,
"third_level_loop_content": third_level_loop_content,
"is_param": is_param,
"is_called": is_called,
"is_call_param": is_call_param,
"in_sum": in_sum,
"in_product": in_product,
"in_binary_operation": in_binary_operation,
"member_accessed": member_accessed}
def counting_condition(value):
"""
This is a custom converter to convert a setting from coala into counting
condition function objects for this bear only.
:param value: An object that can be converted to a list.
:return: A list of functions (counting conditions)
"""
str_list = list(value)
result_list = []
for elem in str_list:
result_list.append(condition_dict.get(elem.lower()))
return result_list
| andreimacavei/coala | bears/codeclone_detection/ClangCountingConditions.py | Python | agpl-3.0 | 15,417 |
"""
Tests for the force_publish management command
"""
from unittest import mock
from django.core.management import CommandError, call_command
from cms.djangoapps.contentstore.management.commands.force_publish import Command
from cms.djangoapps.contentstore.management.commands.utils import get_course_versions
from xmodule.modulestore import ModuleStoreEnum # lint-amnesty, pylint: disable=wrong-import-order
from xmodule.modulestore.tests.django_utils import ModuleStoreTestCase, SharedModuleStoreTestCase # lint-amnesty, pylint: disable=wrong-import-order
from xmodule.modulestore.tests.factories import CourseFactory, ItemFactory # lint-amnesty, pylint: disable=wrong-import-order
class TestForcePublish(SharedModuleStoreTestCase):
"""
Tests for the force_publish management command
"""
@classmethod
def setUpClass(cls):
super().setUpClass()
cls.course = CourseFactory.create(default_store=ModuleStoreEnum.Type.split)
cls.test_user_id = ModuleStoreEnum.UserID.test
cls.command = Command()
def test_no_args(self):
"""
Test 'force_publish' command with no arguments
"""
errstring = "Error: the following arguments are required: course_key"
with self.assertRaisesRegex(CommandError, errstring):
call_command('force_publish')
def test_invalid_course_key(self):
"""
Test 'force_publish' command with invalid course key
"""
errstring = "Invalid course key."
with self.assertRaisesRegex(CommandError, errstring):
call_command('force_publish', 'TestX/TS01')
def test_too_many_arguments(self):
"""
Test 'force_publish' command with more than 2 arguments
"""
errstring = "Error: unrecognized arguments: invalid-arg"
with self.assertRaisesRegex(CommandError, errstring):
call_command('force_publish', str(self.course.id), '--commit', 'invalid-arg')
def test_course_key_not_found(self):
"""
Test 'force_publish' command with non-existing course key
"""
errstring = "Course not found."
with self.assertRaisesRegex(CommandError, errstring):
call_command('force_publish', 'course-v1:org+course+run')
def test_force_publish_non_split(self):
"""
Test 'force_publish' command doesn't work on non split courses
"""
course = CourseFactory.create(default_store=ModuleStoreEnum.Type.mongo)
errstring = 'The owning modulestore does not support this command.'
with self.assertRaisesRegex(CommandError, errstring):
call_command('force_publish', str(course.id))
class TestForcePublishModifications(ModuleStoreTestCase):
"""
Tests for the force_publish management command that modify the courseware
during the test.
"""
def setUp(self):
super().setUp()
self.course = CourseFactory.create(default_store=ModuleStoreEnum.Type.split)
self.test_user_id = ModuleStoreEnum.UserID.test
self.command = Command()
def test_force_publish(self):
"""
Test 'force_publish' command
"""
# Add some changes to course
chapter = ItemFactory.create(category='chapter', parent_location=self.course.location)
self.store.create_child(
self.test_user_id,
chapter.location,
'html',
block_id='html_component'
)
# verify that course has changes.
self.assertTrue(self.store.has_changes(self.store.get_item(self.course.location)))
# get draft and publish branch versions
versions = get_course_versions(str(self.course.id))
draft_version = versions['draft-branch']
published_version = versions['published-branch']
# verify that draft and publish point to different versions
self.assertNotEqual(draft_version, published_version)
with mock.patch('cms.djangoapps.contentstore.management.commands.force_publish.query_yes_no') as patched_yes_no:
patched_yes_no.return_value = True
# force publish course
call_command('force_publish', str(self.course.id), '--commit')
# verify that course has no changes
self.assertFalse(self.store.has_changes(self.store.get_item(self.course.location)))
# get new draft and publish branch versions
versions = get_course_versions(str(self.course.id))
new_draft_version = versions['draft-branch']
new_published_version = versions['published-branch']
# verify that the draft branch didn't change while the published branch did
self.assertEqual(draft_version, new_draft_version)
self.assertNotEqual(published_version, new_published_version)
# verify that draft and publish point to same versions now
self.assertEqual(new_draft_version, new_published_version)
| edx/edx-platform | cms/djangoapps/contentstore/management/commands/tests/test_force_publish.py | Python | agpl-3.0 | 4,997 |
# -*- coding: utf-8 -*-
##############################################################################
#
# OpenERP, Open Source Business Applications
# Copyright (c) 2012-TODAY OpenERP S.A. <http://openerp.com>
#
# This program is free software: you can redistribute it and/or modify
# it under the terms of the GNU Affero General Public License as
# published by the Free Software Foundation, either version 3 of the
# License, or (at your option) any later version.
#
# This program is distributed in the hope that it will be useful,
# but WITHOUT ANY WARRANTY; without even the implied warranty of
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
# GNU Affero General Public License for more details.
#
# You should have received a copy of the GNU Affero General Public License
# along with this program. If not, see <http://www.gnu.org/licenses/>.
#
##############################################################################
from openerp.addons.mail.tests.common import TestMail
from openerp.exceptions import AccessError
from openerp.osv.orm import except_orm
from openerp.tools.misc import mute_logger
class test_portal(TestMail):
def setUp(self):
super(test_portal, self).setUp()
cr, uid = self.cr, self.uid
# Find Portal group
group_portal = self.registry('ir.model.data').get_object(cr, uid, 'base', 'group_portal')
self.group_portal_id = group_portal.id
# Create Chell (portal user)
self.user_chell_id = self.res_users.create(cr, uid, {'name': 'Chell Gladys', 'login': 'chell', 'email': '[email protected]', 'groups_id': [(6, 0, [self.group_portal_id])]})
self.user_chell = self.res_users.browse(cr, uid, self.user_chell_id)
self.partner_chell_id = self.user_chell.partner_id.id
# Create a PigsPortal group
self.group_port_id = self.mail_group.create(cr, uid,
{'name': 'PigsPortal', 'public': 'groups', 'group_public_id': self.group_portal_id},
{'mail_create_nolog': True})
# Set an email address for the user running the tests, used as Sender for outgoing mails
self.res_users.write(cr, uid, uid, {'email': 'test@localhost'})
@mute_logger('openerp.addons.base.ir.ir_model', 'openerp.osv.orm')
def test_00_mail_access_rights(self):
""" Test basic mail_message and mail_group access rights for portal users. """
cr, uid = self.cr, self.uid
mail_compose = self.registry('mail.compose.message')
# Prepare group: Pigs and PigsPortal
pigs_msg_id = self.mail_group.message_post(cr, uid, self.group_pigs_id, body='Message')
port_msg_id = self.mail_group.message_post(cr, uid, self.group_port_id, body='Message')
# Do: Chell browses Pigs -> ko, employee group
chell_pigs = self.mail_group.browse(cr, self.user_chell_id, self.group_pigs_id)
with self.assertRaises(except_orm):
trigger_read = chell_pigs.name
# Do: Chell posts a message on Pigs, crash because can not write on group or is not in the followers
with self.assertRaises(AccessError):
self.mail_group.message_post(cr, self.user_chell_id, self.group_pigs_id, body='Message')
# Do: Chell is added into Pigs followers and browse it -> ok for messages, ko for partners (no read permission)
self.mail_group.message_subscribe_users(cr, uid, [self.group_pigs_id], [self.user_chell_id])
chell_pigs = self.mail_group.browse(cr, self.user_chell_id, self.group_pigs_id)
trigger_read = chell_pigs.name
for message in chell_pigs.message_ids:
trigger_read = message.subject
for partner in chell_pigs.message_follower_ids:
if partner.id == self.partner_chell_id:
# Chell can read her own partner record
continue
with self.assertRaises(except_orm):
trigger_read = partner.name
# Do: Chell comments Pigs, ok because he is now in the followers
self.mail_group.message_post(cr, self.user_chell_id, self.group_pigs_id, body='I love Pigs')
# Do: Chell creates a mail.compose.message record on Pigs, because he uses the wizard
compose_id = mail_compose.create(cr, self.user_chell_id,
{'subject': 'Subject', 'body': 'Body text', 'partner_ids': []},
{'default_composition_mode': 'comment', 'default_model': 'mail.group', 'default_res_id': self.group_pigs_id})
mail_compose.send_mail(cr, self.user_chell_id, [compose_id])
# Do: Chell replies to a Pigs message using the composer
compose_id = mail_compose.create(cr, self.user_chell_id,
{'subject': 'Subject', 'body': 'Body text'},
{'default_composition_mode': 'reply', 'default_parent_id': pigs_msg_id})
mail_compose.send_mail(cr, self.user_chell_id, [compose_id])
# Do: Chell browses PigsPortal -> ok because groups security, ko for partners (no read permission)
chell_port = self.mail_group.browse(cr, self.user_chell_id, self.group_port_id)
trigger_read = chell_port.name
for message in chell_port.message_ids:
trigger_read = message.subject
for partner in chell_port.message_follower_ids:
with self.assertRaises(except_orm):
trigger_read = partner.name
def test_10_mail_invite(self):
cr, uid = self.cr, self.uid
mail_invite = self.registry('mail.wizard.invite')
base_url = self.registry('ir.config_parameter').get_param(cr, uid, 'web.base.url', default='')
# Carine Poilvache, with email, should receive emails for comments and emails
partner_carine_id = self.res_partner.create(cr, uid, {'name': 'Carine Poilvache', 'email': 'c@c'})
# Do: create a mail_wizard_invite, validate it
self._init_mock_build_email()
context = {'default_res_model': 'mail.group', 'default_res_id': self.group_pigs_id}
mail_invite_id = mail_invite.create(cr, uid, {'partner_ids': [(4, partner_carine_id)], 'send_mail': True}, context)
mail_invite.add_followers(cr, uid, [mail_invite_id])
# Test: Pigs followers should contain Admin and Bert
group_pigs = self.mail_group.browse(cr, uid, self.group_pigs_id)
follower_ids = [follower.id for follower in group_pigs.message_follower_ids]
self.assertEqual(set(follower_ids), set([self.partner_admin_id, partner_carine_id]), 'Pigs followers after invite is incorrect')
# Test: partner must have been prepared for signup
partner_carine = self.res_partner.browse(cr, uid, partner_carine_id)
self.assertTrue(partner_carine.signup_valid, 'partner has not been prepared for signup')
self.assertTrue(base_url in partner_carine.signup_url, 'signup url is incorrect')
self.assertTrue(cr.dbname in partner_carine.signup_url, 'signup url is incorrect')
self.assertTrue(partner_carine.signup_token in partner_carine.signup_url, 'signup url is incorrect')
# Test: (pretend to) send email and check subject, body
self.assertEqual(len(self._build_email_kwargs_list), 1, 'sent email number incorrect, should be only for Bert')
for sent_email in self._build_email_kwargs_list:
self.assertEqual(sent_email.get('subject'), 'Invitation to follow Discussion group: Pigs',
'invite: subject of invitation email is incorrect')
self.assertIn('Administrator invited you to follow Discussion group document: Pigs', sent_email.get('body'),
'invite: body of invitation email is incorrect')
self.assertTrue(partner_carine.signup_url in sent_email.get('body'),
'invite: body of invitation email does not contain signup url')
def test_20_notification_url(self):
""" Tests designed to test the URL added in notification emails. """
cr, uid, group_pigs = self.cr, self.uid, self.group_pigs
# Partner data
partner_raoul = self.res_partner.browse(cr, uid, self.partner_raoul_id)
partner_bert_id = self.res_partner.create(cr, uid, {'name': 'bert'})
partner_bert = self.res_partner.browse(cr, uid, partner_bert_id)
# Mail data
mail_mail_id = self.mail_mail.create(cr, uid, {'state': 'exception'})
mail = self.mail_mail.browse(cr, uid, mail_mail_id)
# Test: link for nobody -> None
url = self.mail_mail._get_partner_access_link(cr, uid, mail)
self.assertEqual(url, None,
'notification email: mails not send to a specific partner should not have any URL')
# Test: link for partner -> signup URL
url = self.mail_mail._get_partner_access_link(cr, uid, mail, partner=partner_bert)
self.assertIn(partner_bert.signup_url, url,
'notification email: mails send to a not-user partner should contain the signup URL')
# Test: link for user -> signin
url = self.mail_mail._get_partner_access_link(cr, uid, mail, partner=partner_raoul)
self.assertIn('action=mail.action_mail_redirect', url,
'notification email: link should contain the redirect action')
self.assertIn('login=%s' % partner_raoul.user_ids[0].login, url,
'notification email: link should contain the user login')
@mute_logger('openerp.addons.mail.mail_thread', 'openerp.osv.orm')
def test_21_inbox_redirection(self):
""" Tests designed to test the inbox redirection of emails notification URLs. """
cr, uid, user_admin, group_pigs = self.cr, self.uid, self.user_admin, self.group_pigs
model, act_id = self.ir_model_data.get_object_reference(cr, uid, 'mail', 'action_mail_inbox_feeds')
model, port_act_id = self.ir_model_data.get_object_reference(cr, uid, 'portal', 'action_mail_inbox_feeds_portal')
# Data: post a message on pigs
msg_id = self.group_pigs.message_post(body='My body', partner_ids=[self.partner_bert_id, self.partner_chell_id], type='comment', subtype='mail.mt_comment')
# No specific parameters -> should redirect to Inbox
action = self.mail_thread.message_redirect_action(cr, self.user_raoul_id, {'params': {}})
self.assertEqual(action.get('type'), 'ir.actions.client',
'URL redirection: action without parameters should redirect to client action Inbox')
self.assertEqual(action.get('id'), act_id,
'URL redirection: action without parameters should redirect to client action Inbox')
# Bert has read access to Pigs -> should redirect to form view of Pigs
action = self.mail_thread.message_redirect_action(cr, self.user_raoul_id, {'params': {'message_id': msg_id}})
self.assertEqual(action.get('type'), 'ir.actions.act_window',
'URL redirection: action with message_id for read-accredited user should redirect to Pigs')
self.assertEqual(action.get('res_id'), group_pigs.id,
'URL redirection: action with message_id for read-accredited user should redirect to Pigs')
# Bert has no read access to Pigs -> should redirect to Inbox
action = self.mail_thread.message_redirect_action(cr, self.user_bert_id, {'params': {'message_id': msg_id}})
self.assertEqual(action.get('type'), 'ir.actions.client',
'URL redirection: action without parameters should redirect to client action Inbox')
self.assertEqual(action.get('id'), act_id,
'URL redirection: action without parameters should redirect to client action Inbox')
# Chell has no read access to pigs -> should redirect to Portal Inbox
action = self.mail_thread.message_redirect_action(cr, self.user_chell_id, {'params': {'message_id': msg_id}})
self.assertEqual(action.get('type'), 'ir.actions.client',
'URL redirection: action without parameters should redirect to client action Inbox')
self.assertEqual(action.get('id'), port_act_id,
'URL redirection: action without parameters should redirect to client action Inbox')
def test_30_message_read(self):
cr, uid, group_port_id = self.cr, self.uid, self.group_port_id
# Data: custom subtypes
mt_group_public_id = self.mail_message_subtype.create(cr, uid, {'name': 'group_public', 'description': 'Group changed'})
self.ir_model_data.create(cr, uid, {'name': 'mt_group_public', 'model': 'mail.message.subtype', 'module': 'mail', 'res_id': mt_group_public_id})
# Data: post messages with various subtypes
msg1_id = self.mail_group.message_post(cr, uid, group_port_id, body='Body1', type='comment', subtype='mail.mt_comment')
msg2_id = self.mail_group.message_post(cr, uid, group_port_id, body='Body2', type='comment', subtype='mail.mt_group_public')
msg3_id = self.mail_group.message_post(cr, uid, group_port_id, body='Body3', type='comment', subtype='mail.mt_comment')
msg4_id = self.mail_group.message_post(cr, uid, group_port_id, body='Body4', type='comment')
# msg5_id = self.mail_group.message_post(cr, uid, group_port_id, body='Body5', type='notification')
# Do: Chell search messages: should not see internal notes (comment without subtype)
msg_ids = self.mail_message.search(cr, self.user_chell_id, [('model', '=', 'mail.group'), ('res_id', '=', group_port_id)])
self.assertEqual(set(msg_ids), set([msg1_id, msg2_id, msg3_id]),
'mail_message: portal user has access to messages he should not read')
# Do: Chell read messages she can read
self.mail_message.read(cr, self.user_chell_id, msg_ids, ['body', 'type', 'subtype_id'])
# Do: Chell read a message she should not be able to read
with self.assertRaises(except_orm):
self.mail_message.read(cr, self.user_chell_id, [msg4_id], ['body', 'type', 'subtype_id'])
| jmesteve/saas3 | openerp/addons/portal/tests/test_portal.py | Python | agpl-3.0 | 14,149 |
# -*- coding: utf-8 -*-
# Copyright(C) 2014 Florent Fourcot
#
# This file is part of weboob.
#
# weboob is free software: you can redistribute it and/or modify
# it under the terms of the GNU Affero General Public License as published by
# the Free Software Foundation, either version 3 of the License, or
# (at your option) any later version.
#
# weboob is distributed in the hope that it will be useful,
# but WITHOUT ANY WARRANTY; without even the implied warranty of
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
# GNU Affero General Public License for more details.
#
# You should have received a copy of the GNU Affero General Public License
# along with weboob. If not, see <http://www.gnu.org/licenses/>.
from datetime import date
from weboob.deprecated.browser import Page
from weboob.capabilities.parcel import Parcel, Event
def update_status(p, status):
if p.status < status:
p.status = status
class TrackPage(Page):
def get_info(self, _id):
p = Parcel(_id)
statustr = self.document.xpath('//tr[@class="bandeauText"]')[0]
status = self.parser.tocleanstring(statustr.xpath('td')[1])
p.info = status
p.status = p.STATUS_UNKNOWN
p.history = []
for i, tr in enumerate(self.document.xpath('//div[@class="mainbloc4Evt"]//tr')):
tds = tr.findall('td')
try:
if tds[0].attrib['class'] != "titrestatutdate2":
continue
except:
continue
ev = Event(i)
ev.location = None
ev.activity = self.parser.tocleanstring(tds[1])
if u"Votre colis a été expédié par votre webmarchand" in ev.activity:
update_status(p, p.STATUS_PLANNED)
elif u"Votre colis est pris en charge par Colis Privé" in ev.activity:
update_status(p, p.STATUS_IN_TRANSIT)
elif u"Votre colis est arrivé sur notre agence régionale" in ev.activity:
update_status(p, p.STATUS_IN_TRANSIT)
elif u"Votre colis est en cours de livraison" in ev.activity:
update_status(p, p.STATUS_IN_TRANSIT)
elif u"Votre colis a été livré" in ev.activity:
update_status(p, p.STATUS_ARRIVED)
ev.date = date(*reversed([int(x) for x in self.parser.tocleanstring(tds[0]).split('/')]))
p.history.append(ev)
try:
datelivre = self.document.xpath('//div[@class="NoInstNoRecla"]')
clean = self.parser.tocleanstring(datelivre[0])
if "Votre colis a déja été livré" in clean:
p.status = p.STATUS_ARRIVED
except:
pass
return p
class ErrorPage(Page):
pass
| laurent-george/weboob | modules/colisprive/pages.py | Python | agpl-3.0 | 2,773 |
# -*- coding: utf-8 -*-
# © 2016 Tecnativa, S.L. - Vicent Cubells
# License AGPL-3.0 or later (http://www.gnu.org/licenses/agpl.html).
| Tecnativa/website | website_odoo_debranding/__init__.py | Python | agpl-3.0 | 136 |
# -*- coding: utf-8 -*-
##############################################################################
#
# OpenERP, Open Source Management Solution
# Copyright (C) 2004-2010 Tiny SPRL (<http://tiny.be>).
#
# This program is free software: you can redistribute it and/or modify
# it under the terms of the GNU Affero General Public License as
# published by the Free Software Foundation, either version 3 of the
# License, or (at your option) any later version.
#
# This program is distributed in the hope that it will be useful,
# but WITHOUT ANY WARRANTY; without even the implied warranty of
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
# GNU Affero General Public License for more details.
#
# You should have received a copy of the GNU Affero General Public License
# along with this program. If not, see <http://www.gnu.org/licenses/>.
#
##############################################################################
import time
from report import report_sxw
from osv import osv
import pooler
from tools.translate import _
class product_pricelist(report_sxw.rml_parse):
def __init__(self, cr, uid, name, context):
super(product_pricelist, self).__init__(cr, uid, name, context=context)
self.pricelist=False
self.quantity=[]
self.localcontext.update({
'time': time,
'get_pricelist': self._get_pricelist,
'get_currency': self._get_currency,
'get_currency_symbol': self._get_currency_symbol,
'get_categories': self._get_categories,
'get_price': self._get_price,
'get_titles': self._get_titles,
})
def _get_titles(self,form):
lst = []
vals = {}
qtys = 1
for i in range(1,6):
if form['qty'+str(i)]!=0:
vals['qty'+str(qtys)] = str(form['qty'+str(i)]) + ' units'
qtys += 1
lst.append(vals)
return lst
def _set_quantity(self,form):
for i in range(1,6):
q = 'qty%d'%i
if form[q] >0 and form[q] not in self.quantity:
self.quantity.append(form[q])
else:
self.quantity.append(0)
return True
def _get_pricelist(self, pricelist_id):
pool = pooler.get_pool(self.cr.dbname)
pricelist = pool.get('product.pricelist').read(self.cr, self.uid, [pricelist_id], ['name'], context=self.localcontext)[0]
return pricelist['name']
def _get_currency(self, pricelist_id):
pool = pooler.get_pool(self.cr.dbname)
pricelist = pool.get('product.pricelist').read(self.cr, self.uid, [pricelist_id], ['currency_id'], context=self.localcontext)[0]
return pricelist['currency_id'][1]
def _get_currency_symbol(self, pricelist_id):
pool = pooler.get_pool(self.cr.dbname)
pricelist = pool.get('product.pricelist').read(self.cr, self.uid, [pricelist_id], ['currency_id'], context=self.localcontext)[0]
symbol = pool.get('res.currency').read(self.cr, self.uid, [pricelist['currency_id'][0]], ['symbol'], context=self.localcontext)[0]
return symbol['symbol'] or ''
def _get_categories(self, products,form):
cat_ids=[]
res=[]
self.pricelist = form['price_list']
self._set_quantity(form)
pool = pooler.get_pool(self.cr.dbname)
pro_ids=[]
for product in products:
pro_ids.append(product.id)
if product.categ_id.id not in cat_ids:
cat_ids.append(product.categ_id.id)
cats = pool.get('product.category').name_get(self.cr, self.uid, cat_ids, context=self.localcontext)
if not cats:
return res
for cat in cats:
product_ids=pool.get('product.product').search(self.cr, self.uid, [('id', 'in', pro_ids), ('categ_id', '=', cat[0])], context=self.localcontext)
products = []
for product in pool.get('product.product').read(self.cr, self.uid, product_ids, ['name', 'code'], context=self.localcontext):
val = {
'id':product['id'],
'name':product['name'],
'code':product['code']
}
i = 1
for qty in self.quantity:
if qty == 0:
val['qty'+str(i)] = 0.0
else:
val['qty'+str(i)]=self._get_price(self.pricelist, product['id'], qty)
i += 1
products.append(val)
res.append({'name':cat[1],'products': products})
return res
def _get_price(self,pricelist_id, product_id,qty):
sale_price_digits = self.get_digits(dp='Sale Price')
pool = pooler.get_pool(self.cr.dbname)
price_dict = pool.get('product.pricelist').price_get(self.cr, self.uid, [pricelist_id], product_id, qty, context=self.localcontext)
if price_dict[pricelist_id]:
price = self.formatLang(price_dict[pricelist_id], digits=sale_price_digits)
else:
res = pool.get('product.product').read(self.cr, self.uid, [product_id])
price = self.formatLang(res[0]['list_price'], digits=sale_price_digits)
return price
report_sxw.report_sxw('report.product.pricelist','product.product','addons/product/report/product_pricelist.rml',parser=product_pricelist)
# vim:expandtab:smartindent:tabstop=4:softtabstop=4:shiftwidth=4:
| Johnzero/erp | openerp/addons/product/report/product_pricelist.py | Python | agpl-3.0 | 5,509 |
"""
This config file extends the test environment configuration
so that we can run the lettuce acceptance tests.
"""
# We intentionally define lots of variables that aren't used, and
# want to import all variables from base settings files
# pylint: disable=W0401, W0614
from .test import *
from .sauce import *
# You need to start the server in debug mode,
# otherwise the browser will not render the pages correctly
DEBUG = True
# Output Django logs to a file
import logging
logging.basicConfig(filename=TEST_ROOT / "log" / "lms_acceptance.log", level=logging.ERROR)
import os
from random import choice, randint
import string
def seed():
return os.getppid()
# Use the mongo store for acceptance tests
DOC_STORE_CONFIG = {
'host': 'localhost',
'db': 'acceptance_xmodule',
'collection': 'acceptance_modulestore_%s' % seed(),
}
modulestore_options = {
'default_class': 'xmodule.hidden_module.HiddenDescriptor',
'fs_root': TEST_ROOT / "data",
'render_template': 'edxmako.shortcuts.render_to_string',
}
MODULESTORE = {
'default': {
'ENGINE': 'xmodule.modulestore.mixed.MixedModuleStore',
'OPTIONS': {
'mappings': {},
'stores': {
'default': {
'ENGINE': 'xmodule.modulestore.mongo.MongoModuleStore',
'DOC_STORE_CONFIG': DOC_STORE_CONFIG,
'OPTIONS': modulestore_options
}
}
}
}
}
MODULESTORE['direct'] = MODULESTORE['default']
CONTENTSTORE = {
'ENGINE': 'xmodule.contentstore.mongo.MongoContentStore',
'DOC_STORE_CONFIG': {
'host': 'localhost',
'db': 'acceptance_xcontent_%s' % seed(),
}
}
# Set this up so that rake lms[acceptance] and running the
# harvest command both use the same (test) database
# which they can flush without messing up your dev db
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.sqlite3',
'NAME': TEST_ROOT / "db" / "test_edx.db",
'TEST_NAME': TEST_ROOT / "db" / "test_edx.db",
}
}
TRACKING_BACKENDS.update({
'mongo': {
'ENGINE': 'track.backends.mongodb.MongoBackend'
}
})
# Enable asset pipeline
# Our fork of django-pipeline uses `PIPELINE` instead of `PIPELINE_ENABLED`
# PipelineFinder is explained here: http://django-pipeline.readthedocs.org/en/1.1.24/storages.html
PIPELINE = True
STATICFILES_FINDERS += ('pipeline.finders.PipelineFinder', )
BULK_EMAIL_DEFAULT_FROM_EMAIL = "[email protected]"
# Forums are disabled in test.py to speed up unit tests, but we do not have
# per-test control for acceptance tests
FEATURES['ENABLE_DISCUSSION_SERVICE'] = True
# Use the auto_auth workflow for creating users and logging them in
FEATURES['AUTOMATIC_AUTH_FOR_TESTING'] = True
# Enable fake payment processing page
FEATURES['ENABLE_PAYMENT_FAKE'] = True
# Enable email on the instructor dash
FEATURES['ENABLE_INSTRUCTOR_EMAIL'] = True
FEATURES['REQUIRE_COURSE_EMAIL_AUTH'] = False
# Don't actually send any requests to Software Secure for student identity
# verification.
FEATURES['AUTOMATIC_VERIFY_STUDENT_IDENTITY_FOR_TESTING'] = True
# Configure the payment processor to use the fake processing page
# Since both the fake payment page and the shoppingcart app are using
# the same settings, we can generate this randomly and guarantee
# that they are using the same secret.
RANDOM_SHARED_SECRET = ''.join(
choice(string.letters + string.digits + string.punctuation)
for x in range(250)
)
CC_PROCESSOR['CyberSource']['SHARED_SECRET'] = RANDOM_SHARED_SECRET
CC_PROCESSOR['CyberSource']['MERCHANT_ID'] = "edx"
CC_PROCESSOR['CyberSource']['SERIAL_NUMBER'] = "0123456789012345678901"
CC_PROCESSOR['CyberSource']['PURCHASE_ENDPOINT'] = "/shoppingcart/payment_fake"
# HACK
# Setting this flag to false causes imports to not load correctly in the lettuce python files
# We do not yet understand why this occurs. Setting this to true is a stopgap measure
USE_I18N = True
FEATURES['ENABLE_FEEDBACK_SUBMISSION'] = True
FEEDBACK_SUBMISSION_EMAIL = '[email protected]'
# Include the lettuce app for acceptance testing, including the 'harvest' django-admin command
INSTALLED_APPS += ('lettuce.django',)
LETTUCE_APPS = ('courseware', 'instructor',)
# Lettuce appears to have a bug that causes it to search
# `instructor_task` when we specify the `instructor` app.
# This causes some pretty cryptic errors as lettuce tries
# to parse files in `instructor_task` as features.
# As a quick workaround, explicitly exclude the `instructor_task` app.
LETTUCE_AVOID_APPS = ('instructor_task',)
LETTUCE_BROWSER = os.environ.get('LETTUCE_BROWSER', 'chrome')
# Where to run: local, saucelabs, or grid
LETTUCE_SELENIUM_CLIENT = os.environ.get('LETTUCE_SELENIUM_CLIENT', 'local')
SELENIUM_GRID = {
'URL': 'http://127.0.0.1:4444/wd/hub',
'BROWSER': LETTUCE_BROWSER,
}
#####################################################################
# See if the developer has any local overrides.
try:
from .private import * # pylint: disable=F0401
except ImportError:
pass
# Because an override for where to run will affect which ports to use,
# set these up after the local overrides.
# Configure XQueue interface to use our stub XQueue server
XQUEUE_INTERFACE = {
"url": "http://127.0.0.1:{0:d}".format(XQUEUE_PORT),
"django_auth": {
"username": "lms",
"password": "***REMOVED***"
},
"basic_auth": ('anant', 'agarwal'),
}
# Point the URL used to test YouTube availability to our stub YouTube server
YOUTUBE_TEST_URL = "http://127.0.0.1:{0}/test_youtube/".format(YOUTUBE_PORT)
| pelikanchik/edx-platform | lms/envs/acceptance.py | Python | agpl-3.0 | 5,619 |
"""Deprecated import support. Auto-generated by import_shims/generate_shims.sh."""
# pylint: disable=redefined-builtin,wrong-import-position,wildcard-import,useless-suppression,line-too-long
from import_shims.warn import warn_deprecated_import
warn_deprecated_import('contentstore.tests.test_import_draft_order', 'cms.djangoapps.contentstore.tests.test_import_draft_order')
from cms.djangoapps.contentstore.tests.test_import_draft_order import *
| eduNEXT/edunext-platform | import_shims/studio/contentstore/tests/test_import_draft_order.py | Python | agpl-3.0 | 449 |
# Copyright 2013-2020 Lawrence Livermore National Security, LLC and other
# Spack Project Developers. See the top-level COPYRIGHT file for details.
#
# SPDX-License-Identifier: (Apache-2.0 OR MIT)
from spack import *
class RSpatial(RPackage):
"""spatial: Functions for Kriging and Point Pattern Analysis"""
homepage = "https://cloud.r-project.org/package=spatial"
url = "https://cloud.r-project.org/src/contrib/spatial_7.3-11.tar.gz"
list_url = "https://cloud.r-project.org/src/contrib/Archive/spatial"
version('7.3-11', sha256='624448d2ac22e1798097d09fc5dc4605908a33f490b8ec971fc6ea318a445c11')
depends_on('[email protected]:', type=('build', 'run'))
depends_on('r-mass', type=('build', 'run'))
| iulian787/spack | var/spack/repos/builtin/packages/r-spatial/package.py | Python | lgpl-2.1 | 725 |
# Copyright 2013-2020 Lawrence Livermore National Security, LLC and other
# Spack Project Developers. See the top-level COPYRIGHT file for details.
#
# SPDX-License-Identifier: (Apache-2.0 OR MIT)
from spack import *
from os import listdir
class Cntk1bitsgd(Package):
"""CNTK1bitSGD is the header-only
1-bit stochastic gradient descent (1bit-SGD) library for
the Computational Network Toolkit (CNTK)."""
homepage = "https://github.com/CNTK-components/CNTK1bitSGD"
git = "https://github.com/CNTK-components/CNTK1bitSGD.git"
version('master')
version('c8b77d', commit='c8b77d6e325a4786547b27624890276c1483aed1')
def install(self, spec, prefix):
mkdirp(prefix.include)
for file in listdir('.'):
if file.endswith('.h'):
install(file, prefix.include)
| iulian787/spack | var/spack/repos/builtin/packages/cntk1bitsgd/package.py | Python | lgpl-2.1 | 833 |
#!/usr/bin/python -u
# Dedicated Control handler script for OpenLieroX
# (http://openlierox.sourceforge.net)
import time
import os
import sys
import threading
import traceback
import math
import dedicated_control_io as io
setvar = io.setvar
formatExceptionInfo = io.formatExceptionInfo
import dedicated_control_ranking as ranking
import dedicated_control_handler as hnd
adminCommandHelp_Preset = None
parseAdminCommand_Preset = None
userCommandHelp_Preset = None
parseUserCommand_Preset = None
kickedUsers = {} # IP and timeout for it
def adminCommandHelp(wormid):
io.privateMsg(wormid, "Admin help:")
io.privateMsg(wormid, "%skick wormID [time] [reason]" % cfg.ADMIN_PREFIX)
io.privateMsg(wormid, "%sban wormID [reason]" % cfg.ADMIN_PREFIX)
io.privateMsg(wormid, "%smute wormID" % cfg.ADMIN_PREFIX)
io.privateMsg(wormid, "%spreset presetName" % cfg.ADMIN_PREFIX)
io.privateMsg(wormid, "%smod modName (or part of name)" % cfg.ADMIN_PREFIX)
io.privateMsg(wormid, "%smap mapName" % cfg.ADMIN_PREFIX)
io.privateMsg(wormid, "%slt loadingTime" % cfg.ADMIN_PREFIX)
io.privateMsg(wormid, "%steam wormID teamID (0123 or brgy)" % cfg.ADMIN_PREFIX)
io.privateMsg(wormid, "%sstart - start game now" % cfg.ADMIN_PREFIX)
io.privateMsg(wormid, "%sstop - go to lobby" % cfg.ADMIN_PREFIX)
io.privateMsg(wormid, "%spause - pause ded script" % cfg.ADMIN_PREFIX)
io.privateMsg(wormid, "%sunpause - resume ded script" % cfg.ADMIN_PREFIX)
io.privateMsg(wormid, "%ssetvar varname value" % cfg.ADMIN_PREFIX)
io.privateMsg(wormid, "%sauthorize wormID" % cfg.ADMIN_PREFIX)
if adminCommandHelp_Preset:
adminCommandHelp_Preset(wormid)
# Admin interface
def parseAdminCommand(wormid,message):
global kickedUsers
try: # Do not check on msg size or anything, exception handling is further down
if (not message.startswith(cfg.ADMIN_PREFIX)):
return False # normal chat
cmd = message.split(" ")[0]
cmd = cmd.replace(cfg.ADMIN_PREFIX,"",1).lower() #Remove the prefix
if wormid >= 0:
io.messageLog("%i:%s issued %s" % (wormid,hnd.worms[wormid].Name,cmd.replace(cfg.ADMIN_PREFIX,"",1)),io.LOG_ADMIN)
else:
io.messageLog("ded admin issued %s" % cmd, io.LOG_USRCMD)
# Unnecesary to split multiple times, this saves CPU.
params = message.split(" ")[1:]
if cmd == "help":
adminCommandHelp(wormid)
elif cmd == "kick":
kickTime = cfg.VOTING_KICK_TIME
if len(params) > 1: # Time for kick
kickTime = float(params[1])
wormIP = io.getWormIP(int( params[0] )).split(":")[0]
if wormIP != "127.0.0.1":
kickedUsers[ wormIP ] = time.time() + kickTime*60
if len(params) > 2: # Given some reason
io.kickWorm( int( params[0] ), " ".join(params[2:]) )
else:
io.kickWorm( int( params[0] ) )
elif cmd == "ban":
if len(params) > 1: # Given some reason
io.banWorm( int( params[0] ), " ".join(params[1:]) )
else:
io.banWorm( int( params[0] ) )
elif cmd == "mute":
io.muteWorm( int( params[0] ) )
elif cmd == "preset":
preset = -1
for p in range(len(hnd.availablePresets)):
if hnd.availablePresets[p].lower().find(params[0].lower()) != -1:
preset = p
break
if preset == -1:
io.privateMsg(wormid,"Invalid preset, available presets: " + ", ".join(hnd.availablePresets))
else:
hnd.selectPreset( Preset = hnd.availablePresets[preset] )
elif cmd == "mod":
mod = ""
for m in io.listMods():
if m.lower().find(" ".join(params[0:]).lower()) != -1:
mod = m
break
if mod == "":
io.privateMsg(wormid,"Invalid mod, available mods: " + ", ".join(io.listMods()))
else:
hnd.selectPreset( Mod = mod )
elif cmd == "map":
level = ""
for l in io.listMaps():
if l.lower().find(" ".join(params[0:]).lower()) != -1:
level = l
break
if level == "":
io.privateMsg(wormid,"Invalid map, available maps: " + ", ".join(io.listMaps()))
else:
hnd.selectPreset( Level = level )
elif cmd == "lt":
hnd.selectPreset( LT = int(params[0]) )
elif cmd == "start":
io.startGame()
elif cmd == "stop":
io.gotoLobby()
elif cmd == "pause":
io.privateMsg(wormid,"Ded script paused")
hnd.scriptPaused = True
elif cmd == "unpause":
io.privateMsg(wormid,"Ded script continues")
hnd.scriptPaused = False
elif cmd == "setvar":
io.setvar(params[0], " ".join(params[1:])) # In case value contains spaces
elif cmd == "authorize":
try:
wormID = int(params[0])
if not hnd.worms[wormID].isAdmin:
hnd.worms[wormID].isAdmin = True
io.authorizeWorm(wormID)
io.messageLog( "Worm %i (%s) added to admins by %i (%s)" % (wormID,hnd.worms[wormID].Name,wormid,hnd.worms[wormid].Name),io.LOG_INFO)
io.privateMsg(wormID, "%s made you admin! Type %shelp for commands" % (hnd.worms[wormid].Name,cfg.ADMIN_PREFIX))
io.privateMsg(wormid, "%s added to admins." % hnd.worms[wormID].Name)
except KeyError:
io.messageLog("parseAdminCommand: Our local copy of wormses doesn't match the real list.",io.LOG_ERROR)
elif parseAdminCommand_Preset and parseAdminCommand_Preset(wormid, cmd, params):
pass
else:
raise Exception, "Invalid admin command"
except: # All python classes derive from main "Exception", but confused me, this has the same effect.
if wormid >= 0:
io.privateMsg(wormid, "Invalid admin command")
io.messageLog(formatExceptionInfo(),io.LOG_ERROR) #Helps to fix errors
return False
return True
# User interface
def userCommandHelp(wormid):
if cfg.ALLOW_TEAM_CHANGE:
msg = "%steam [b/r" % (cfg.USER_PREFIX)
if cfg.MAX_TEAMS >= 3:
msg += "/g"
if cfg.MAX_TEAMS >= 4:
msg += "/y"
msg += "] - set your team"
io.privateMsg(wormid, msg + " - set your team")
if cfg.RANKING:
io.privateMsg(wormid, "%stoprank - display the best players" % cfg.USER_PREFIX )
io.privateMsg(wormid, "%srank [name] - display your or other player rank" % cfg.USER_PREFIX )
io.privateMsg(wormid, "%sranktotal - display the number of players in the ranking" % cfg.USER_PREFIX )
if cfg.VOTING:
io.privateMsg(wormid, "%skick wormID - add vote to kick player etc" % cfg.ADMIN_PREFIX)
io.privateMsg(wormid, "%smute wormID - add vote" % cfg.ADMIN_PREFIX)
io.privateMsg(wormid, "%smod modName (or part of name) - add vote" % cfg.ADMIN_PREFIX)
io.privateMsg(wormid, "%smap mapName - add vote" % cfg.ADMIN_PREFIX)
io.privateMsg(wormid, "%slt loadingTime - add vote" % cfg.ADMIN_PREFIX)
io.privateMsg(wormid, "%sstart - start game now" % cfg.ADMIN_PREFIX)
io.privateMsg(wormid, "%sstop - go to lobby" % cfg.ADMIN_PREFIX)
io.privateMsg(wormid, "%sy / %sn - vote yes / no" % (cfg.ADMIN_PREFIX, cfg.ADMIN_PREFIX) )
if userCommandHelp_Preset:
userCommandHelp_Preset(wormid)
voteCommand = None
voteTime = 0
votePoster = -1
voteDescription = ""
def addVote( command, poster, description ):
global voteCommand, voteTime, votePoster, voteDescription
if time.time() - voteTime < cfg.VOTING_TIME:
io.privateMsg(poster, "Previous vote still running, " + str(int( cfg.VOTING_TIME + voteTime - time.time() )) + " seconds left")
return
if time.time() - hnd.worms[poster].FailedVoteTime < cfg.VOTING_TIME * 2:
io.privateMsg(poster, "You cannot add vote for " + str(int( cfg.VOTING_TIME * 2 + hnd.worms[poster].FailedVoteTime - time.time() )) + " seconds")
return
voteCommand = command
for w in hnd.worms.keys():
hnd.worms[w].Voted = 0
votePoster = poster
hnd.worms[poster].Voted = 1
voteTime = time.time()
voteDescription = description
recheckVote()
def recheckVote(verbose = True):
global voteCommand, voteTime, votePoster, voteDescription
global kickedUsers
if not voteCommand:
return
voteCount = 0
notVoted = 0
if cfg.VOTING_COUNT_NEGATIVE:
for w in hnd.worms.keys():
voteCount += hnd.worms[w].Voted
if hnd.worms[w].Voted == 0:
notVoted += 1
else:
for w in hnd.worms.keys():
if hnd.worms[w].Voted == 1:
voteCount += 1
else:
notVoted += 1
humanWormCount = len(hnd.worms) - len(io.getComputerWormList())
needVoices = int( math.ceil( humanWormCount * cfg.VOTING_PERCENT / 100.0 ) )
if voteCount >= needVoices or (time.time() - voteTime >= cfg.VOTING_TIME and cfg.VOTING_AUTO_ACCEPT):
try:
exec(voteCommand)
except:
io.messageLog(formatExceptionInfo(),io.LOG_ERROR) #Helps to fix errors
voteCommand = None
voteTime = 0
return
if time.time() - voteTime >= cfg.VOTING_TIME or needVoices - voteCount > notVoted:
voteCommand = None
voteTime = 0
io.chatMsg("Vote failed: " + voteDescription )
if ( votePoster in hnd.worms.keys() ) and ( hnd.worms[votePoster].Voted == 1 ): # Check if worm left and another worm joined with same ID
hnd.worms[votePoster].FailedVoteTime = time.time()
return
if verbose:
io.chatMsg("Vote: " + voteDescription + ", " + str( needVoices - voteCount ) + " voices to go, " +
str(int( cfg.VOTING_TIME + voteTime - time.time() )) + ( " seconds, say %sy or %sn" % ( cfg.ADMIN_PREFIX, cfg.ADMIN_PREFIX ) ) )
def parseUserCommand(wormid,message):
global kickedUsers
try: # Do not check on msg size or anything, exception handling is further down
if message in [ "y", "n", "start", "stop", "rank", "toprank", "ranktotal" ]:
# Single-word usercommands for faster typing
cmd = message
else:
if (not message.startswith(cfg.USER_PREFIX)):
return False # normal chat
cmd = message.split(" ")[0]
cmd = cmd.replace(cfg.USER_PREFIX,"",1).lower() #Remove the prefix
if wormid >= 0:
io.messageLog("%i:%s issued %s" % (wormid,hnd.worms[wormid].Name,cmd.replace(cfg.USER_PREFIX,"",1)),io.LOG_USRCMD)
else:
io.messageLog("ded admin issued %s" % cmd, io.LOG_USRCMD)
# Unnecesary to split multiple times, this saves CPU.
params = message.split(" ")[1:]
if cmd == "help":
userCommandHelp(wormid)
if cfg.ALLOW_TEAM_CHANGE and cmd == "team":
if not params:
io.privateMsg(wormid, "You need to specify a team" )
raise Exception, "You need to specify a team"
else:
if params[0].lower() == "blue" or params[0].lower() == "b":
io.setWormTeam(wormid, 0)
elif params[0].lower() == "red" or params[0].lower() == "r":
io.setWormTeam(wormid, 1)
elif ( params[0].lower() == "green" or params[0].lower() == "g" ) and cfg.MAX_TEAMS >= 3:
io.setWormTeam(wormid, 2)
elif ( params[0].lower() == "yellow" or params[0].lower() == "y" ) and cfg.MAX_TEAMS >= 4:
io.setWormTeam(wormid, 3)
if cfg.RANKING:
if cmd == "toprank":
ranking.firstRank(wormid)
if cmd == "rank":
if wormid in hnd.worms:
wormName = hnd.worms[wormid].Name
if params:
wormName = " ".join(params)
ranking.myRank(wormName, wormid)
if cmd == "ranktotal":
io.privateMsg(wormid, "There are " + str(len(ranking.rank)) + " players in the ranking.")
if cfg.VOTING:
if cmd == "kick":
kicked = int( params[0] )
if not kicked in hnd.worms.keys():
raise Exception, "Invalid worm ID"
addVote( "io.kickWorm(" + str(kicked) +
", 'You are kicked for " + str(cfg.VOTING_KICK_TIME) + " minutes')",
wormid, "Kick %i: %s" % ( kicked, hnd.worms[kicked].Name ) )
hnd.worms[kicked].Voted = -1
if cmd == "mute":
if not kicked in hnd.worms.keys():
raise Exception, "Invalid worm ID"
kicked = int( params[0] )
addVote( "io.muteWorm(" + str(kicked) +")", wormid, "Mute %i: %s" % ( kicked, hnd.worms[kicked].Name ) )
hnd.worms[kicked].Voted = -1
if cmd == "mod":
# Users are proven to be stupid and can't tell the difference between mod and preset
# so here we're first looking for a preset, and then looking for a mod with the same name if preset not found
# (well, let's call that UI simplification)
preset = -1
for p in range(len(hnd.availablePresets)):
if hnd.availablePresets[p].lower().find(params[0].lower()) != -1:
preset = p
break
if preset != -1:
addVote( 'hnd.selectPreset( Preset = "%s" )' % hnd.availablePresets[preset], wormid, "Preset %s" % hnd.availablePresets[preset] )
else:
mod = ""
for m in io.listMods():
if m.lower().find(" ".join(params[0:]).lower()) != -1:
mod = m
break
if mod == "":
io.privateMsg(wormid,"Invalid mod, available mods: " + ", ".join(hnd.availablePresets) + ", ".join(io.listMods()))
else:
addVote( 'hnd.selectPreset( Mod = "%s" )' % mod, wormid, "Mod %s" % mod )
if cmd == "map":
level = ""
for l in io.listMaps():
if l.lower().find(" ".join(params[0:]).lower()) != -1:
level = l
break
if level == "":
io.privateMsg(wormid,"Invalid map, available maps: " + ", ".join(io.listMaps()))
else:
addVote( 'hnd.selectPreset( Level = "%s" )' % level, wormid, "Map %s" % level )
if cmd == "lt":
addVote( 'hnd.selectPreset( LT = %i )' % int(params[0]), wormid, "Loading time %i" % int(params[0]) )
if cmd == "start":
addVote( 'hnd.lobbyWaitAfterGame = time.time(); hnd.lobbyWaitBeforeGame = time.time()', wormid, "Start game now" )
if cmd == "stop":
addVote( 'io.gotoLobby()', wormid, "Go to lobby" )
if ( cmd == "y" or cmd == "yes" ):
if hnd.worms[wormid].Voted != 1:
hnd.worms[wormid].Voted = 1
recheckVote()
if ( cmd == "n" or cmd == "no" ):
if hnd.worms[wormid].Voted != -1:
hnd.worms[wormid].Voted = -1
recheckVote()
elif parseUserCommand_Preset and parseUserCommand_Preset(wormid, cmd, params):
pass
else:
raise Exception, "Invalid user command"
except: # All python classes derive from main "Exception", but confused me, this has the same effect.
if wormid >= 0:
io.privateMsg(wormid, "Invalid user command - type !help for list of commands")
io.messageLog(formatExceptionInfo(),io.LOG_ERROR) #Helps to fix errors
return False
return True
| ProfessorKaos64/openlierox | share/gamedir/scripts/dedicated_control_usercommands.py | Python | lgpl-2.1 | 13,799 |
from waflib.Build import BuildContext
import subprocess
import os
class dumpconfig(BuildContext):
'''dumps the libs connected to the targets'''
cmd = 'dumpconfig'
fun = 'build'
def execute(self):
self.restore()
if not self.all_envs:
self.load_envs()
self.recurse([self.run_dir])
targetsStr = '--targets=' + self.targets
# Create the output string
output = os.path.join(self.env['PREFIX'], 'include')
output += '\n'
proc = subprocess.Popen(['python', 'waf', 'dumpenv', targetsStr],
stdout=subprocess.PIPE,
universal_newlines=True)
dump = proc.stdout.read().rstrip().split(' ')
# Remove PYTHONDIR and PYTHONARCHDIR
for value in dump:
if 'PYTHONDIR' not in value and 'PYTHONARCHDIR' not in value:
output += value + ' '
output += '\n'
proc = subprocess.Popen(['python', 'waf', 'dumplib-raw', targetsStr],
stdout=subprocess.PIPE,
universal_newlines=True)
dump = proc.stdout.read().rstrip()
if not dump.startswith('No dependencies'):
output += dump
output += '\n'
output += os.path.join(self.env['PREFIX'], 'lib')
output += '\n'
# Write the output to file
f = open(os.path.join(self.env['PREFIX'], 'prebuilt_config.txt'), 'w')
f.write(output)
f.close()
| mdaus/nitro | externals/coda-oss/build/dumpconfig.py | Python | lgpl-3.0 | 1,463 |
#!/usr/bin/env python
"""
Usage: synapse_densities.py --help
Voxelizes and optionally shows synapse densities.
"""
import argparse
import hashlib
import os
import subprocess
__author__ = "Daniel Nachbaur"
__email__ = "[email protected]"
__copyright__ = "Copyright 2016, EPFL/Blue Brain Project"
def _find_executable(executable):
"""
Search for executable in PATH and return result
"""
from distutils import spawn
executable_path = spawn.find_executable(executable)
if not executable_path:
print("Cannot find {0} executable in PATH".format(executable))
return False
return True
def _use_vgl():
is_ssh = 'SSH_CLIENT' in os.environ
is_vglconnect = is_ssh and ('VGL_DISPLAY' in os.environ or
'VGL_CLIENT' in os.environ)
try:
is_ssh_x = is_ssh and 'localhost:' in os.environ['DISPLAY']
except KeyError:
is_ssh_x = False
is_vnc = 'VNCDESKTOP' in os.environ
return is_vglconnect or is_vnc or is_ssh_x
class Launcher(object): # pylint: disable=too-few-public-methods
"""
Launcher for a tool showing synapse density volume.
"""
def __init__(self, args):
"""
Setup volume URI for livre and/or voxelize from args.
"""
self._usevgl = _use_vgl()
self._args = args
self._args.resolution = 1./self._args.resolution
self._volume = 'fivoxsynapses://{config}?resolution={resolution}&'
if args.target:
self._volume += 'target={target}'
self._outname = 'density_{target}'.format(**vars(self._args))
else:
self._volume += 'preTarget={projection[0]}&postTarget={projection[1]}'
self._outname = 'density_{projection[0]}_{projection[1]}'.format(**vars(self._args))
if args.fraction:
self._volume += '&gidFraction={fraction}'
if args.reference:
self._volume += '&reference={reference}'
else:
self._volume += '&extend=200'
if args.datarange:
self._volume += '&inputMin={datarange[0]}&inputMax={datarange[1]}'
self._volume = self._volume.format(**vars(self._args))
if args.verbose:
print(self._volume)
hash_object = hashlib.md5(str(self._volume))
self._outname += "_{0}.nrrd".format(hash_object.hexdigest()[:7])
self._outname = os.path.abspath(self._outname)
def launch(self):
"""
Launch livre or voxelize and/or paraview.
"""
if os.path.exists(self._outname):
print("Reusing previously generated volume {0}".format(self._outname))
if self._args.tool == 'livre':
if os.path.exists(self._outname):
volume = "raw://{0}".format(self._outname)
else:
volume = self._volume
args = ['livre', '--volume', volume, '--max-lod 0']
if self._usevgl:
args.insert(0, 'vglrun')
subprocess.call(args)
if self._args.tool == 'voxelize' or self._args.tool == 'paraview':
if not os.path.exists(self._outname):
subprocess.call(['voxelize', '--volume', self._volume,
'-o', self._outname])
if self._args.tool == 'paraview':
args = ['paraview', self._outname]
if self._usevgl:
args.insert(0, 'vglrun')
subprocess.call(args)
def main():
"""
Find the available tools and launch them according to user input.
"""
tools = ['livre', 'paraview', 'voxelize']
executables = list()
for tool in tools:
if _find_executable(tool):
executables.append(tool)
if not executables:
print("Cannot find any tools in PATH")
exit()
parser = argparse.ArgumentParser(
description="A tool for synapse densities visualization",
epilog="If paraview or voxelize was launched, it creates a "
"'density_{target}_{hash}.nrrd' volume file in the current "
"directory which will be reused if this tool was called again "
"with the same parameters.")
parser.add_argument("tool", choices=executables,
help="Tool to use for volume generation/visualization")
parser.add_argument("-c", "--config", metavar='<BlueConfig>',
help="path to blue config file", required=True)
group = parser.add_mutually_exclusive_group(required=True)
group.add_argument("-t", "--target", metavar='<target>',
help="target for afferent synapses")
group.add_argument('-p', "--projection", nargs=2, metavar=('pre', 'post'),
help="targets for synaptic projections")
parser.add_argument("-f", "--fraction", metavar='<GID fraction>',
help="fraction of GIDs to use [0,1]")
parser.add_argument("-d", "--datarange", nargs=2, metavar=('min', 'max'),
help="data range to use")
parser.add_argument("-r", "--resolution", metavar="<micrometer/voxel>", default=16, type=float,
help="resolution in micrometer/voxel, default 16")
parser.add_argument("--reference", metavar='<path to volume>', default="",
help="path to reference volume for size and resolution setup")
parser.add_argument("-v", "--verbose", action="store_true",
help="Print volume URI")
args = parser.parse_args()
launcher = Launcher(args)
launcher.launch()
if __name__ == "__main__":
main()
| BlueBrain/Fivox | apps/synapseDensities/synapse_densities.py | Python | lgpl-3.0 | 5,654 |
import unittest
from construct import *
from construct.text import *
class NodeAdapter(Adapter):
def __init__(self, factory, subcon):
Adapter.__init__(self, subcon)
self.factory = factory
def _decode(self, obj, context):
return self.factory(obj)
#===============================================================================
# AST nodes
#===============================================================================
class Node(Container):
def __init__(self, name, **kw):
Container.__init__(self)
self.name = name
for k, v in kw.iteritems():
setattr(self, k, v)
def accept(self, visitor):
return getattr(visitor, "visit_%s" % self.name)(self)
def binop_node(obj):
lhs, rhs = obj
if rhs is None:
return lhs
else:
op, rhs = rhs
return Node("binop", lhs=lhs, op=op, rhs=rhs)
def literal_node(value):
return Node("literal", value = value)
#===============================================================================
# concrete grammar
#===============================================================================
ws = Whitespace()
term = IndexingAdapter(
Sequence("term",
ws,
Select("term",
NodeAdapter(literal_node, DecNumber("number")),
IndexingAdapter(
Sequence("subexpr",
Literal("("),
LazyBound("expr", lambda: expr),
Literal(")")
),
index = 0
),
),
ws,
),
index = 0
)
def OptSeq(name, *args):
return Optional(Sequence(name, *args))
expr1 = NodeAdapter(binop_node,
Sequence("expr1",
term,
OptSeq("rhs",
CharOf("op", "*/"),
LazyBound("rhs", lambda: expr1)
),
)
)
expr2 = NodeAdapter(binop_node,
Sequence("expr2",
expr1,
OptSeq("rhs",
CharOf("op", "+-"),
LazyBound("rhs", lambda: expr2)
),
)
)
expr = expr2
#===============================================================================
# evaluation visitor
#===============================================================================
class EvalVisitor(object):
def visit_literal(self, obj):
return obj.value
def visit_binop(self, obj):
lhs = obj.lhs.accept(self)
op = obj.op
rhs = obj.rhs.accept(self)
if op == "+":
return lhs + rhs
elif op == "-":
return lhs - rhs
elif op == "*":
return lhs * rhs
elif op == "/":
return lhs / rhs
else:
raise ValueError("invalid op", op)
ev = EvalVisitor()
class TestSomethingSomething(unittest.TestCase):
def test_that_one_thing(self):
node = expr.parse("2*3+4")
self.assertEqual(node.name, "binop")
self.assertEqual(node.op, "+")
self.assertEqual(node.rhs.name, "literal")
self.assertEqual(node.rhs.value, 4)
self.assertEqual(node.lhs.name, "binop")
self.assertEqual(node.lhs.op, "*")
self.assertEqual(node.lhs.rhs.name, "literal")
self.assertEqual(node.lhs.rhs.value, 3)
self.assertEqual(node.lhs.lhs.name, "literal")
self.assertEqual(node.lhs.lhs.value, 2)
def test_that_other_thing(self):
node = expr.parse("2*(3+4)")
self.assertEqual(node.name, "binop")
self.assertEqual(node.op, "*")
self.assertEqual(node.rhs.name, "binop")
self.assertEqual(node.rhs.op, "+")
self.assertEqual(node.rhs.rhs.name, "literal")
self.assertEqual(node.rhs.rhs.value, 4)
self.assertEqual(node.rhs.lhs.name, "literal")
self.assertEqual(node.rhs.lhs.value, 3)
self.assertEqual(node.lhs.name, "literal")
self.assertEqual(node.lhs.value, 2)
| Joev-/HoNCore | honcore/lib/construct/tests/test_ast.py | Python | unlicense | 3,908 |
# Copyright 2016 The TensorFlow Authors. All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# ==============================================================================
"""Py2TF compiles Python code into equivalent TensorFlow code.
Equivalent here means that they have the same effect when executed.
"""
from __future__ import absolute_import
from __future__ import division
from __future__ import print_function
from tensorflow.contrib.py2tf import utils
from tensorflow.contrib.py2tf.impl.api import convert
from tensorflow.contrib.py2tf.impl.api import graph_ready
from tensorflow.contrib.py2tf.impl.api import to_code
from tensorflow.contrib.py2tf.impl.api import to_graph
from tensorflow.contrib.py2tf.pyct.transformer import PyFlowParseError
from tensorflow.python.util.all_util import remove_undocumented
_allowed_symbols = [
'to_graph', 'to_code', 'convert', 'graph_ready', 'utils', 'PyFlowParseError'
]
remove_undocumented(__name__, _allowed_symbols)
| nolanliou/tensorflow | tensorflow/contrib/py2tf/__init__.py | Python | apache-2.0 | 1,489 |
# Copyright (c) 2018 PaddlePaddle Authors. All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
import unittest
import numpy as np
from op_test import OpTest
def crop(data, offsets, crop_shape):
def indexOf(shape, index):
result = []
for dim in reversed(shape):
result.append(index % dim)
index = index / dim
return result[::-1]
result = []
for i, value in enumerate(data.flatten()):
index = indexOf(data.shape, i)
selected = True
if len(index) == len(offsets):
for j, offset in enumerate(offsets):
selected = selected and index[j] >= offset and index[
j] < crop_shape[j] + offset
if selected:
result.append(value)
return np.array(result).reshape(crop_shape)
class TestCropOp(OpTest):
def setUp(self):
self.op_type = "crop"
self.crop_by_input = False
self.attrs = {}
self.initTestCase()
self.attrs['offsets'] = self.offsets
if self.crop_by_input:
self.inputs = {
'X': np.random.random(self.x_shape).astype("float32"),
'Y': np.random.random(self.crop_shape).astype("float32")
}
else:
self.attrs['shape'] = self.crop_shape
self.inputs = {
'X': np.random.random(self.x_shape).astype("float32"),
}
self.outputs = {
'Out': crop(self.inputs['X'], self.offsets, self.crop_shape)
}
def initTestCase(self):
self.x_shape = (8, 8)
self.crop_shape = (2, 2)
self.offsets = [1, 2]
def test_check_output(self):
self.check_output()
def test_check_grad_normal(self):
self.check_grad(['X'], 'Out', max_relative_error=0.006)
class TestCase1(TestCropOp):
def initTestCase(self):
self.x_shape = (16, 8, 32)
self.crop_shape = [2, 2, 3]
self.offsets = [1, 5, 3]
class TestCase2(TestCropOp):
def initTestCase(self):
self.x_shape = (4, 8)
self.crop_shape = [4, 8]
self.offsets = [0, 0]
class TestCase3(TestCropOp):
def initTestCase(self):
self.x_shape = (4, 8, 16)
self.crop_shape = [2, 2, 3]
self.offsets = [1, 5, 3]
self.crop_by_input = True
class TestCase4(TestCropOp):
def initTestCase(self):
self.x_shape = (4, 4)
self.crop_shape = [4, 4]
self.offsets = [0, 0]
self.crop_by_input = True
if __name__ == '__main__':
unittest.main()
| lcy-seso/Paddle | python/paddle/fluid/tests/unittests/test_crop_op.py | Python | apache-2.0 | 3,089 |
#!/usr/bin/env python
# -- Content-Encoding: UTF-8 --
"""
Instance manager class definition
:author: Thomas Calmant
:copyright: Copyright 2016, Thomas Calmant
:license: Apache License 2.0
:version: 0.6.4
..
Copyright 2016 Thomas Calmant
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
"""
# Standard library
import logging
import threading
import traceback
# Pelix
from pelix.constants import FrameworkException
# iPOPO constants
import pelix.ipopo.constants as constants
import pelix.ipopo.handlers.constants as handlers_const
# iPOPO beans
from pelix.ipopo.contexts import ComponentContext
# ------------------------------------------------------------------------------
# Module version
__version_info__ = (0, 6, 4)
__version__ = ".".join(str(x) for x in __version_info__)
# Documentation strings format
__docformat__ = "restructuredtext en"
# ------------------------------------------------------------------------------
class StoredInstance(object):
"""
Represents a component instance
"""
# Try to reduce memory footprint (stored instances)
__slots__ = ('bundle_context', 'context', 'factory_name', 'instance',
'name', 'state', '_controllers_state', '_handlers',
'_ipopo_service', '_lock', '_logger', 'error_trace',
'__all_handlers')
INVALID = 0
""" This component has been invalidated """
VALID = 1
""" This component has been validated """
KILLED = 2
""" This component has been killed """
VALIDATING = 3
""" This component is currently validating """
ERRONEOUS = 4
""" This component has failed while validating """
def __init__(self, ipopo_service, context, instance, handlers):
"""
Sets up the instance object
:param ipopo_service: The iPOPO service that instantiated this
component
:param context: The component context
:param instance: The component instance
:param handlers: The list of handlers associated to this component
"""
assert isinstance(context, ComponentContext)
# The logger
self._logger = logging.getLogger(
'-'.join(("InstanceManager", context.name)))
# The lock
self._lock = threading.RLock()
# The iPOPO service
self._ipopo_service = ipopo_service
# Component context
self.context = context
# The instance name
self.name = self.context.name
# Factory name
self.factory_name = self.context.get_factory_name()
# Component instance
self.instance = instance
# Set the instance state
self.state = StoredInstance.INVALID
# Stack track of validation error
self.error_trace = None
# Store the bundle context
self.bundle_context = self.context.get_bundle_context()
# The controllers state dictionary
self._controllers_state = {}
# Handlers: kind -> [handlers]
self._handlers = {}
self.__all_handlers = set(handlers)
for handler in handlers:
kinds = handler.get_kinds()
if kinds:
for kind in kinds:
self._handlers.setdefault(kind, []).append(handler)
def __repr__(self):
"""
String representation
"""
return self.__str__()
def __str__(self):
"""
String representation
"""
return "StoredInstance(Name={0}, State={1})" \
.format(self.name, self.state)
def check_event(self, event):
"""
Tests if the given service event must be handled or ignored, based
on the state of the iPOPO service and on the content of the event.
:param event: A service event
:return: True if the event can be handled, False if it must be ignored
"""
with self._lock:
if self.state == StoredInstance.KILLED:
# This call may have been blocked by the internal state lock,
# ignore it
return False
return self.__safe_handlers_callback('check_event', event)
def bind(self, dependency, svc, svc_ref):
"""
Called by a dependency manager to inject a new service and update the
component life cycle.
"""
with self._lock:
self.__set_binding(dependency, svc, svc_ref)
self.check_lifecycle()
def update(self, dependency, svc, svc_ref, old_properties,
new_value=False):
"""
Called by a dependency manager when the properties of an injected
dependency have been updated.
:param dependency: The dependency handler
:param svc: The injected service
:param svc_ref: The reference of the injected service
:param old_properties: Previous properties of the dependency
:param new_value: If True, inject the new value of the handler
"""
with self._lock:
self.__update_binding(dependency, svc, svc_ref, old_properties,
new_value)
self.check_lifecycle()
def unbind(self, dependency, svc, svc_ref):
"""
Called by a dependency manager to remove an injected service and to
update the component life cycle.
"""
with self._lock:
# Invalidate first (if needed)
self.check_lifecycle()
# Call unbind() and remove the injection
self.__unset_binding(dependency, svc, svc_ref)
# Try a new configuration
if self.update_bindings():
self.check_lifecycle()
def get_controller_state(self, name):
"""
Retrieves the state of the controller with the given name
:param name: The name of the controller
:return: The value of the controller
:raise KeyError: No value associated to this controller
"""
return self._controllers_state[name]
def set_controller_state(self, name, value):
"""
Sets the state of the controller with the given name
:param name: The name of the controller
:param value: The new value of the controller
"""
with self._lock:
self._controllers_state[name] = value
self.__safe_handlers_callback('on_controller_change', name, value)
def update_property(self, name, old_value, new_value):
"""
Handles a property changed event
:param name: The changed property name
:param old_value: The previous property value
:param new_value: The new property value
"""
with self._lock:
self.__safe_handlers_callback('on_property_change', name,
old_value, new_value)
def update_hidden_property(self, name, old_value, new_value):
"""
Handles an hidden property changed event
:param name: The changed property name
:param old_value: The previous property value
:param new_value: The new property value
"""
with self._lock:
self.__safe_handlers_callback(
'on_hidden_property_change', name, old_value, new_value)
def get_handlers(self, kind=None):
"""
Retrieves the handlers of the given kind. If kind is None, all handlers
are returned.
:param kind: The kind of the handlers to return
:return: A list of handlers, or an empty list
"""
with self._lock:
if kind is not None:
try:
return self._handlers[kind][:]
except KeyError:
return []
return self.__all_handlers.copy()
def check_lifecycle(self):
"""
Tests if the state of the component must be updated, based on its own
state and on the state of its dependencies
"""
with self._lock:
# Validation flags
was_valid = (self.state == StoredInstance.VALID)
can_validate = self.state not in (StoredInstance.VALIDATING,
StoredInstance.VALID)
# Test the validity of all handlers
handlers_valid = self.__safe_handlers_callback(
'is_valid', break_on_false=True)
if was_valid and not handlers_valid:
# A dependency is missing
self.invalidate(True)
elif can_validate and handlers_valid \
and self._ipopo_service.running:
# We're all good
self.validate(True)
def update_bindings(self):
"""
Updates the bindings of the given component
:return: True if the component can be validated
"""
with self._lock:
all_valid = True
for handler in self.get_handlers(handlers_const.KIND_DEPENDENCY):
# Try to bind
self.__safe_handler_callback(handler, 'try_binding')
# Update the validity flag
all_valid &= self.__safe_handler_callback(
handler, 'is_valid', only_boolean=True, none_as_true=True)
return all_valid
def start(self):
"""
Starts the handlers
"""
with self._lock:
self.__safe_handlers_callback('start')
def retry_erroneous(self, properties_update):
"""
Removes the ERRONEOUS state from a component and retries a validation
:param properties_update: A dictionary to update component properties
:return: The new state of the component
"""
with self._lock:
if self.state != StoredInstance.ERRONEOUS:
# Not in erroneous state: ignore
return self.state
# Update properties
if properties_update:
self.context.properties.update(properties_update)
# Reset state
self.state = StoredInstance.INVALID
self.error_trace = None
# Retry
self.check_lifecycle()
# Check if the component is still erroneous
return self.state
def invalidate(self, callback=True):
"""
Applies the component invalidation.
:param callback: If True, call back the component before the
invalidation
:return: False if the component wasn't valid
"""
with self._lock:
if self.state != StoredInstance.VALID:
# Instance is not running...
return False
# Change the state
self.state = StoredInstance.INVALID
# Call the handlers
self.__safe_handlers_callback('pre_invalidate')
# Call the component
if callback:
self.safe_callback(constants.IPOPO_CALLBACK_INVALIDATE,
self.bundle_context)
# Trigger an "Invalidated" event
self._ipopo_service._fire_ipopo_event(
constants.IPopoEvent.INVALIDATED,
self.factory_name, self.name)
# Call the handlers
self.__safe_handlers_callback('post_invalidate')
return True
def kill(self):
"""
This instance is killed : invalidate it if needed, clean up all members
When this method is called, this StoredInstance object must have
been removed from the registry
:return: True if the component has been killed, False if it already was
"""
with self._lock:
# Already dead...
if self.state == StoredInstance.KILLED:
return False
try:
self.invalidate(True)
except:
self._logger.exception("%s: Error invalidating the instance",
self.name)
# Now that we are nearly clean, be sure we were in a good registry
# state
assert not self._ipopo_service.is_registered_instance(self.name)
# Stop all handlers (can tell to unset a binding)
for handler in self.get_handlers():
results = self.__safe_handler_callback(handler, 'stop')
if results:
try:
for binding in results:
self.__unset_binding(handler, binding[0],
binding[1])
except Exception as ex:
self._logger.exception(
"Error stopping handler '%s': %s", handler, ex)
# Call the handlers
self.__safe_handlers_callback('clear')
# Change the state
self.state = StoredInstance.KILLED
# Trigger the event
self._ipopo_service._fire_ipopo_event(constants.IPopoEvent.KILLED,
self.factory_name, self.name)
# Clean up members
self._handlers.clear()
self.__all_handlers.clear()
self._handlers = None
self.__all_handlers = None
self.context = None
self.instance = None
self._ipopo_service = None
return True
def validate(self, safe_callback=True):
"""
Ends the component validation, registering services
:param safe_callback: If True, calls the component validation callback
:return: True if the component has been validated, else False
:raise RuntimeError: You try to awake a dead component
"""
with self._lock:
if self.state in (StoredInstance.VALID,
StoredInstance.VALIDATING,
StoredInstance.ERRONEOUS):
# No work to do (yet)
return False
if self.state == StoredInstance.KILLED:
raise RuntimeError("{0}: Zombies !".format(self.name))
# Clear the error trace
self.error_trace = None
# Call the handlers
self.__safe_handlers_callback('pre_validate')
if safe_callback:
# Safe call back needed and not yet passed
self.state = StoredInstance.VALIDATING
if not self.safe_callback(constants.IPOPO_CALLBACK_VALIDATE,
self.bundle_context):
# Stop there if the callback failed
self.state = StoredInstance.VALID
self.invalidate(True)
# Consider the component has erroneous
self.state = StoredInstance.ERRONEOUS
return False
# All good
self.state = StoredInstance.VALID
# Call the handlers
self.__safe_handlers_callback('post_validate')
# We may have caused a framework error, so check if iPOPO is active
if self._ipopo_service is not None:
# Trigger the iPOPO event (after the service _registration)
self._ipopo_service._fire_ipopo_event(
constants.IPopoEvent.VALIDATED,
self.factory_name, self.name)
return True
def __callback(self, event, *args, **kwargs):
"""
Calls the registered method in the component for the given event
:param event: An event (IPOPO_CALLBACK_VALIDATE, ...)
:return: The callback result, or None
:raise Exception: Something went wrong
"""
comp_callback = self.context.get_callback(event)
if not comp_callback:
# No registered callback
return True
# Call it
result = comp_callback(self.instance, *args, **kwargs)
if result is None:
# Special case, if the call back returns nothing
return True
return result
def __field_callback(self, field, event, *args, **kwargs):
"""
Calls the registered method in the component for the given field event
:param field: A field name
:param event: An event (IPOPO_CALLBACK_VALIDATE, ...)
:return: The callback result, or None
:raise Exception: Something went wrong
"""
# Get the field callback info
cb_info = self.context.get_field_callback(field, event)
if not cb_info:
# No registered callback
return True
# Extract information
callback, if_valid = cb_info
if if_valid and self.state != StoredInstance.VALID:
# Don't call the method if the component state isn't satisfying
return True
# Call it
result = callback(self.instance, field, *args, **kwargs)
if result is None:
# Special case, if the call back returns nothing
return True
return result
def safe_callback(self, event, *args, **kwargs):
"""
Calls the registered method in the component for the given event,
ignoring raised exceptions
:param event: An event (IPOPO_CALLBACK_VALIDATE, ...)
:return: The callback result, or None
"""
if self.state == StoredInstance.KILLED:
# Invalid state
return None
try:
return self.__callback(event, *args, **kwargs)
except FrameworkException as ex:
# Important error
self._logger.exception("Critical error calling back %s: %s",
self.name, ex)
# Kill the component
self._ipopo_service.kill(self.name)
# Store the exception in case of a validation error
if event == constants.IPOPO_CALLBACK_VALIDATE:
self.error_trace = traceback.format_exc()
if ex.needs_stop:
# Framework must be stopped...
self._logger.error("%s said that the Framework must be "
"stopped.", self.name)
self.bundle_context.get_bundle(0).stop()
return False
except:
self._logger.exception("Component '%s': error calling callback "
"method for event %s", self.name, event)
# Store the exception in case of a validation error
if event == constants.IPOPO_CALLBACK_VALIDATE:
self.error_trace = traceback.format_exc()
return False
def __safe_field_callback(self, field, event, *args, **kwargs):
"""
Calls the registered method in the component for the given event,
ignoring raised exceptions
:param field: Name of the modified field
:param event: A field event (IPOPO_CALLBACK_BIND_FIELD, ...)
:return: The callback result, or None
"""
if self.state == StoredInstance.KILLED:
# Invalid state
return None
try:
return self.__field_callback(field, event, *args, **kwargs)
except FrameworkException as ex:
# Important error
self._logger.exception("Critical error calling back %s: %s",
self.name, ex)
# Kill the component
self._ipopo_service.kill(self.name)
if ex.needs_stop:
# Framework must be stopped...
self._logger.error("%s said that the Framework must be "
"stopped.", self.name)
self.bundle_context.get_bundle(0).stop()
return False
except:
self._logger.exception("Component '%s' : error calling "
"callback method for event %s",
self.name, event)
return False
def __safe_handler_callback(self, handler, method_name, *args, **kwargs):
"""
Calls the given method with the given arguments in the given handler.
Logs exceptions, but doesn't propagate them.
Special arguments can be given in kwargs:
* 'none_as_true': If set to True and the method returned None or
doesn't exist, the result is considered as True.
If set to False, None result is kept as is.
Default is False.
* 'only_boolean': If True, the result can only be True or False, else
the result is the value returned by the method.
Default is False.
:param handler: The handler to call
:param method_name: The name of the method to call
:param args: List of arguments for the method to call
:param kwargs: Dictionary of arguments for the method to call and to
control the call
:return: The method result, or None on error
"""
if handler is None or method_name is None:
return None
# Behavior flags
only_boolean = kwargs.pop('only_boolean', False)
none_as_true = kwargs.pop('none_as_true', False)
# Get the method for each handler
try:
method = getattr(handler, method_name)
except AttributeError:
# Method not found
result = None
else:
try:
# Call it
result = method(*args, **kwargs)
except Exception as ex:
# No result
result = None
# Log error
self._logger.exception("Error calling handler '%s': %s",
handler, ex)
if result is None and none_as_true:
# Consider None (nothing returned) as True
result = True
if only_boolean:
# Convert to a boolean result
return bool(result)
return result
def __safe_handlers_callback(self, method_name, *args, **kwargs):
"""
Calls the given method with the given arguments in all handlers.
Logs exceptions, but doesn't propagate them.
Methods called in handlers must return None, True or False.
Special parameters can be given in kwargs:
* 'exception_as_error': if it is set to True and an exception is raised
by a handler, then this method will return False. By default, this
flag is set to False and exceptions are ignored.
* 'break_on_false': if it set to True, the loop calling the handler
will stop after an handler returned False. By default, this flag
is set to False, and all handlers are called.
:param method_name: Name of the method to call
:param args: List of arguments for the method to call
:param kwargs: Dictionary of arguments for the method to call and the
behavior of the call
:return: True if all handlers returned True (or None), else False
"""
if self.state == StoredInstance.KILLED:
# Nothing to do
return False
# Behavior flags
exception_as_error = kwargs.pop('exception_as_error', False)
break_on_false = kwargs.pop('break_on_false', False)
result = True
for handler in self.get_handlers():
# Get the method for each handler
try:
method = getattr(handler, method_name)
except AttributeError:
# Ignore missing methods
pass
else:
try:
# Call it
res = method(*args, **kwargs)
if res is not None and not res:
# Ignore 'None' results
result = False
except Exception as ex:
# Log errors
self._logger.exception("Error calling handler '%s': %s",
handler, ex)
# We can consider exceptions as errors or ignore them
result &= not exception_as_error
if not handler and break_on_false:
# The loop can stop here
break
return result
def __set_binding(self, dependency, service, reference):
"""
Injects a service in the component
:param dependency: The dependency handler
:param service: The injected service
:param reference: The reference of the injected service
"""
# Set the value
setattr(self.instance, dependency.get_field(), dependency.get_value())
# Call the component back
self.safe_callback(constants.IPOPO_CALLBACK_BIND, service, reference)
self.__safe_field_callback(dependency.get_field(),
constants.IPOPO_CALLBACK_BIND_FIELD,
service, reference)
def __update_binding(self, dependency, service, reference, old_properties,
new_value):
"""
Calls back component binding and field binding methods when the
properties of an injected dependency have been updated.
:param dependency: The dependency handler
:param service: The injected service
:param reference: The reference of the injected service
:param old_properties: Previous properties of the dependency
:param new_value: If True, inject the new value of the handler
"""
if new_value:
# Set the value
setattr(self.instance, dependency.get_field(),
dependency.get_value())
# Call the component back
self.__safe_field_callback(dependency.get_field(),
constants.IPOPO_CALLBACK_UPDATE_FIELD,
service, reference, old_properties)
self.safe_callback(constants.IPOPO_CALLBACK_UPDATE, service,
reference, old_properties)
def __unset_binding(self, dependency, service, reference):
"""
Removes a service from the component
:param dependency: The dependency handler
:param service: The injected service
:param reference: The reference of the injected service
"""
# Call the component back
self.__safe_field_callback(dependency.get_field(),
constants.IPOPO_CALLBACK_UNBIND_FIELD,
service, reference)
self.safe_callback(constants.IPOPO_CALLBACK_UNBIND, service, reference)
# Update the injected field
setattr(self.instance, dependency.get_field(), dependency.get_value())
# Unget the service
self.bundle_context.unget_service(reference)
| isandlaTech/cohorte-devtools | qualifier/deploy/cohorte-home/repo/pelix/ipopo/instance.py | Python | apache-2.0 | 27,681 |
# Copyright (c) 2015-2016 Claudiu Popa <[email protected]>
# Licensed under the GPL: https://www.gnu.org/licenses/old-licenses/gpl-2.0.html
# For details: https://github.com/PyCQA/pylint/blob/master/COPYING
"""JSON reporter"""
from __future__ import absolute_import, print_function
import cgi
import json
import sys
from pylint.interfaces import IReporter
from pylint.reporters import BaseReporter
class JSONReporter(BaseReporter):
"""Report messages and layouts in JSON."""
__implements__ = IReporter
name = 'json'
extension = 'json'
def __init__(self, output=sys.stdout):
BaseReporter.__init__(self, output)
self.messages = []
def handle_message(self, msg):
"""Manage message of different type and in the context of path."""
self.messages.append({
'type': msg.category,
'module': msg.module,
'obj': msg.obj,
'line': msg.line,
'column': msg.column,
'path': msg.path,
'symbol': msg.symbol,
# pylint: disable=deprecated-method; deprecated since 3.2.
'message': cgi.escape(msg.msg or ''),
})
def display_messages(self, layout):
"""Launch layouts display"""
if self.messages:
print(json.dumps(self.messages, indent=4), file=self.out)
def display_reports(self, layout): # pylint: disable=arguments-differ
"""Don't do nothing in this reporter."""
def _display(self, layout):
"""Don't do nothing."""
def register(linter):
"""Register the reporter classes with the linter."""
linter.register_reporter(JSONReporter)
| arju88nair/projectCulminate | venv/lib/python3.5/site-packages/pylint/reporters/json.py | Python | apache-2.0 | 1,662 |
"""Representation of a deCONZ remote or keypad."""
from pydeconz.sensor import (
ANCILLARY_CONTROL_EMERGENCY,
ANCILLARY_CONTROL_FIRE,
ANCILLARY_CONTROL_INVALID_CODE,
ANCILLARY_CONTROL_PANIC,
AncillaryControl,
Switch,
)
from homeassistant.const import (
CONF_DEVICE_ID,
CONF_EVENT,
CONF_ID,
CONF_UNIQUE_ID,
CONF_XY,
)
from homeassistant.core import callback
from homeassistant.helpers.dispatcher import async_dispatcher_connect
from homeassistant.util import slugify
from .const import CONF_ANGLE, CONF_GESTURE, LOGGER, NEW_SENSOR
from .deconz_device import DeconzBase
CONF_DECONZ_EVENT = "deconz_event"
CONF_DECONZ_ALARM_EVENT = "deconz_alarm_event"
SUPPORTED_DECONZ_ALARM_EVENTS = {
ANCILLARY_CONTROL_EMERGENCY,
ANCILLARY_CONTROL_FIRE,
ANCILLARY_CONTROL_INVALID_CODE,
ANCILLARY_CONTROL_PANIC,
}
async def async_setup_events(gateway) -> None:
"""Set up the deCONZ events."""
@callback
def async_add_sensor(sensors=gateway.api.sensors.values()):
"""Create DeconzEvent."""
for sensor in sensors:
if not gateway.option_allow_clip_sensor and sensor.type.startswith("CLIP"):
continue
if (
sensor.type not in Switch.ZHATYPE + AncillaryControl.ZHATYPE
or sensor.uniqueid in {event.unique_id for event in gateway.events}
):
continue
if sensor.type in Switch.ZHATYPE:
new_event = DeconzEvent(sensor, gateway)
elif sensor.type in AncillaryControl.ZHATYPE:
new_event = DeconzAlarmEvent(sensor, gateway)
gateway.hass.async_create_task(new_event.async_update_device_registry())
gateway.events.append(new_event)
gateway.config_entry.async_on_unload(
async_dispatcher_connect(
gateway.hass, gateway.async_signal_new_device(NEW_SENSOR), async_add_sensor
)
)
async_add_sensor()
@callback
def async_unload_events(gateway) -> None:
"""Unload all deCONZ events."""
for event in gateway.events:
event.async_will_remove_from_hass()
gateway.events.clear()
class DeconzEvent(DeconzBase):
"""When you want signals instead of entities.
Stateless sensors such as remotes are expected to generate an event
instead of a sensor entity in hass.
"""
def __init__(self, device, gateway):
"""Register callback that will be used for signals."""
super().__init__(device, gateway)
self._device.register_callback(self.async_update_callback)
self.device_id = None
self.event_id = slugify(self._device.name)
LOGGER.debug("deCONZ event created: %s", self.event_id)
@property
def device(self):
"""Return Event device."""
return self._device
@callback
def async_will_remove_from_hass(self) -> None:
"""Disconnect event object when removed."""
self._device.remove_callback(self.async_update_callback)
@callback
def async_update_callback(self, force_update=False):
"""Fire the event if reason is that state is updated."""
if (
self.gateway.ignore_state_updates
or "state" not in self._device.changed_keys
):
return
data = {
CONF_ID: self.event_id,
CONF_UNIQUE_ID: self.serial,
CONF_EVENT: self._device.state,
}
if self.device_id:
data[CONF_DEVICE_ID] = self.device_id
if self._device.gesture is not None:
data[CONF_GESTURE] = self._device.gesture
if self._device.angle is not None:
data[CONF_ANGLE] = self._device.angle
if self._device.xy is not None:
data[CONF_XY] = self._device.xy
self.gateway.hass.bus.async_fire(CONF_DECONZ_EVENT, data)
async def async_update_device_registry(self) -> None:
"""Update device registry."""
if not self.device_info:
return
device_registry = (
await self.gateway.hass.helpers.device_registry.async_get_registry()
)
entry = device_registry.async_get_or_create(
config_entry_id=self.gateway.config_entry.entry_id, **self.device_info
)
self.device_id = entry.id
class DeconzAlarmEvent(DeconzEvent):
"""Alarm control panel companion event when user interacts with a keypad."""
@callback
def async_update_callback(self, force_update=False):
"""Fire the event if reason is new action is updated."""
if (
self.gateway.ignore_state_updates
or "action" not in self._device.changed_keys
or self._device.action not in SUPPORTED_DECONZ_ALARM_EVENTS
):
return
data = {
CONF_ID: self.event_id,
CONF_UNIQUE_ID: self.serial,
CONF_DEVICE_ID: self.device_id,
CONF_EVENT: self._device.action,
}
self.gateway.hass.bus.async_fire(CONF_DECONZ_ALARM_EVENT, data)
| Danielhiversen/home-assistant | homeassistant/components/deconz/deconz_event.py | Python | apache-2.0 | 5,074 |
Subsets and Splits