repo
stringlengths 26
115
| file
stringlengths 54
212
| language
stringclasses 2
values | license
stringclasses 16
values | content
stringlengths 19
1.07M
|
---|---|---|---|---|
https://github.com/fsr/rust-lessons | https://raw.githubusercontent.com/fsr/rust-lessons/master/src/slides.typ | typst | #let section = state("section", none)
#let subslide = counter("subslide")
#let logical-slide = counter("logical-slide")
#let repetitions = counter("repetitions")
#let global-theme = state("global-theme", none)
#let new-section(name) = section.update(name)
#let _slides-cover(mode, body) = {
if mode == "invisible" {
hide(body)
} else if mode == "transparent" {
text(gray.lighten(50%), body)
} else {
panic("Illegal cover mode: " + mode)
}
}
#let _parse-subslide-indices(s) = {
let parts = s.split(",").map(p => p.trim())
let parse-part(part) = {
let match-until = part.match(regex("^-([[:digit:]]+)$"))
let match-beginning = part.match(regex("^([[:digit:]]+)-$"))
let match-range = part.match(regex("^([[:digit:]]+)-([[:digit:]]+)$"))
let match-single = part.match(regex("^([[:digit:]]+)$"))
if match-until != none {
let parsed = int(match-until.captures.first())
// assert(parsed > 0, "parsed idx is non-positive")
( until: parsed )
} else if match-beginning != none {
let parsed = int(match-beginning.captures.first())
// assert(parsed > 0, "parsed idx is non-positive")
( beginning: parsed )
} else if match-range != none {
let parsed-first = int(match-range.captures.first())
let parsed-last = int(match-range.captures.last())
// assert(parsed-first > 0, "parsed idx is non-positive")
// assert(parsed-last > 0, "parsed idx is non-positive")
( beginning: parsed-first, until: parsed-last )
} else if match-single != none {
let parsed = int(match-single.captures.first())
// assert(parsed > 0, "parsed idx is non-positive")
parsed
} else {
panic("failed to parse visible slide idx:" + part)
}
}
parts.map(parse-part)
}
#let _check-visible(idx, visible-subslides) = {
if type(visible-subslides) == "integer" {
idx == visible-subslides
} else if type(visible-subslides) == "array" {
visible-subslides.any(s => _check-visible(idx, s))
} else if type(visible-subslides) == "string" {
let parts = _parse-subslide-indices(visible-subslides)
_check-visible(idx, parts)
} else if type(visible-subslides) == "dictionary" {
let lower-okay = if "beginning" in visible-subslides {
visible-subslides.beginning <= idx
} else {
true
}
let upper-okay = if "until" in visible-subslides {
visible-subslides.until >= idx
} else {
true
}
lower-okay and upper-okay
} else {
panic("you may only provide a single integer, an array of integers, or a string")
}
}
#let _last-required-subslide(visible-subslides) = {
if type(visible-subslides) == "integer" {
visible-subslides
} else if type(visible-subslides) == "array" {
calc.max(..visible-subslides.map(s => _last-required-subslide(s)))
} else if type(visible-subslides) == "string" {
let parts = _parse-subslide-indices(visible-subslides)
_last-required-subslide(parts)
} else if type(visible-subslides) == "dictionary" {
let last = 0
if "beginning" in visible-subslides {
last = calc.max(last, visible-subslides.beginning)
}
if "until" in visible-subslides {
last = calc.max(last, visible-subslides.until)
}
last
} else {
panic("you may only provide a single integer, an array of integers, or a string")
}
}
#let _conditional-display(visible-subslides, reserve-space, mode, body) = {
repetitions.update(rep => calc.max(rep, _last-required-subslide(visible-subslides)))
locate( loc => {
if _check-visible(subslide.at(loc).first(), visible-subslides) {
body
} else if reserve-space {
_slides-cover(mode, body)
}
})
}
#let uncover(visible-subslides, mode: "invisible", body) = {
_conditional-display(visible-subslides, true, mode, body)
}
#let only(visible-subslides, body) = {
_conditional-display(visible-subslides, false, "doesn't even matter", body)
}
#let begin(subslidestart, body) = {
uncover((beginning: subslidestart), body)
}
#let one-by-one(start: 1, mode: "invisible", ..children) = {
repetitions.update(rep => calc.max(rep, start + children.pos().len() - 1))
for (idx, child) in children.pos().enumerate() {
uncover((beginning: start + idx), mode: mode, child)
}
}
#let alternatives(start: 1, position: bottom + left, ..children) = {
repetitions.update(rep => calc.max(rep, start + children.pos().len() - 1))
style(styles => {
let sizes = children.pos().map(c => measure(c, styles))
let max-width = calc.max(..sizes.map(sz => sz.width))
let max-height = calc.max(..sizes.map(sz => sz.height))
for (idx, child) in children.pos().enumerate() {
only(start + idx, box(
width: max-width,
height: max-height,
align(position, child)
))
}
})
}
#let line-by-line(start: 1, mode: "invisible", body) = {
let items = if repr(body.func()) == "sequence" {
body.children
} else {
( body, )
}
let idx = start
for item in items {
if repr(item.func()) != "space" {
uncover((beginning: idx), mode: mode, item)
idx += 1
} else {
item
}
}
}
#let pause(beginning, mode: "invisible") = body => {
uncover((beginning: beginning), mode: mode, body)
}
#let slides-default-theme(color: teal) = data => {
let title-slide = {
align(center + horizon)[
#block(
stroke: ( y: 1mm + color ),
inset: 1em,
breakable: false,
[
#text(1.3em)[*#data.title*] \
#{
if data.subtitle != none {
parbreak()
text(.9em)[#data.subtitle]
}
}
]
)
#set text(size: .8em)
#grid(
columns: (1fr,) * calc.min(data.authors.len(), 3),
column-gutter: 1em,
row-gutter: 1em,
..data.authors
)
#v(1em)
#data.date
]
}
let default(slide-info, body) = {
let decoration(position, body) = {
let border = 1mm + color
let strokes = (
header: ( bottom: border ),
footer: ( top: border )
)
block(
stroke: strokes.at(position),
width: 100%,
inset: .3em,
text(.5em, body)
)
}
// header
decoration("header", section.display())
if "title" in slide-info {
block(
width: 100%, inset: (x: 2em), breakable: false,
outset: 0em,
heading(level: 1, slide-info.title)
)
}
v(1fr)
block(
width: 100%, inset: (x: 2em), breakable: false, outset: 0em,
body
)
v(2fr)
// footer
decoration("footer")[
#data.short-authors #h(10fr)
#data.short-title #h(1fr)
#data.date #h(10fr)
#logical-slide.display()
]
}
let wake-up(slide-info, body) = {
block(
width: 100%, height: 100%, inset: 2em, breakable: false, outset: 0em,
fill: color,
text(size: 1.5em, fill: white, {v(1fr); body; v(1fr)})
)
}
let action(slide-info, body) = {
let action-color = orange
let decoration(position, body) = {
let border = 1mm + action-color
let strokes = (
header: ( bottom: border ),
footer: ( top: border )
)
block(
stroke: strokes.at(position),
width: 100%,
inset: .3em,
text(.5em, body)
)
}
// header
decoration("header", section.display())
if "title" in slide-info {
let action-heading = text(fill: action-color)[#slide-info.title]
block(
width: 100%, inset: (x: 2em), breakable: false,
outset: 0em,
heading(level: 1, action-heading)
)
}
v(1fr)
block(
width: 100%, inset: (x: 2em), breakable: false, outset: 0em,
body
)
v(2fr)
// footer
decoration("footer")[
#data.short-authors #h(10fr)
#data.short-title #h(1fr)
#data.date #h(10fr)
#logical-slide.display()
]
}
(
title-slide: title-slide,
variants: (
"default": default,
"wake up": wake-up,
"action": action,
),
)
}
#let slide(
max-repetitions: 10,
theme-variant: "default",
override-theme: none,
..kwargs,
body
) = {
pagebreak(weak: true)
logical-slide.step()
locate( loc => {
subslide.update(1)
repetitions.update(1)
let slide-content = global-theme.at(loc).variants.at(theme-variant)
if override-theme != none {
slide-content = override-theme
}
let slide-info = kwargs.named()
for _ in range(max-repetitions) {
locate( loc-inner => {
let curr-subslide = subslide.at(loc-inner).first()
if curr-subslide <= repetitions.at(loc-inner).first() {
if curr-subslide > 1 { pagebreak(weak: true) }
slide-content(slide-info, body)
}
})
subslide.step()
}
})
}
#let slides(
title: none,
authors: none,
subtitle: none,
short-title: none,
short-authors: none,
date: none,
theme: slides-default-theme(),
typography: (:),
body
) = {
if "text-size" not in typography {
typography.text-size = 20pt
}
if "paper" not in typography {
typography.paper = "presentation-16-9"
}
if "text-font" not in typography {
typography.text-font = ("Inria Sans")
}
if "math-font" not in typography {
typography.math-font = ("GFS Neohellenic Math")
}
if "raw-font" not in typography {
typography.raw-font = ("Iosevka")
}
set text(
size: typography.text-size,
font: typography.text-font,
)
show math.equation: set text(font: typography.math-font)
show raw: set text(font: typography.raw-font)
set page(
paper: typography.paper,
margin: 0pt,
)
let data = (
title: title,
authors: if type(authors) == "array" {
authors
} else if type(authors) in ("string", "content") {
(authors, )
} else {
panic("authors must be an array, string, or content.")
},
subtitle: subtitle,
short-title: short-title,
short-authors: short-authors,
date: date,
)
let the-theme = theme(data)
global-theme.update(the-theme)
the-theme.title-slide
body
}
#let two-grid(
right-width: 40%,
left,
body
) = {
let cell = rect.with(
inset: 8pt,
stroke: none,
width: 100%,
height: 70%,
radius: 6pt,
)
grid(
columns: (1fr, right-width),
rows: (auto),
gutter: 3pt,
cell()[#left],
cell()[#body],
)
}
|
|
https://github.com/0x1B05/nju_os | https://raw.githubusercontent.com/0x1B05/nju_os/main/lecture_notes/content/04_Python建模操作系统.typ | typst | #import "../template.typ": *
#pagebreak()
= Python 建模操作系统
== 理解操作系统的新途径
=== 回顾:程序/硬件的状态机模型
计算机软件
- 状态机 (C/汇编)
- 允许执行特殊指令 (syscall) 请求操作系统
- 操作系统 = API + 对象
计算机硬件
- “无情执行指令的机器”
- 从 CPU Reset 状态开始执行 Firmware 代码
- 操作系统 = C 程序
=== 一个大胆的想法
无论是软件还是硬件,都是状态机
- 而状态和状态的迁移是可以 “画” 出来的!
- 理论上说,只需要两个 API
- `dump_state()` - 获取当前程序状态
- `single_step()` - 执行一步
- `gdb` 不就是做这个的吗!
=== GDB 检查状态的缺陷
太复杂
- 状态太多 (指令数很多)
- 状态太大 (很多库函数状态)
简化:把复杂的东西分解成简单的东西
- 在《操作系统》课上,简化是非常重要的主题, 否则容易迷失在细节的海洋中,
一些具体的例子:
- 只关注系统调用 (strace)
- `strace`把指令简化为两种,一种计算指令(不关心),另一种是 system call(关心)的指令,
这种简化可以看到软件和操作系统边界在发生什么.
- Makefile 的命令日志
- strace/Makefile 日志的清理
=== 一个想法:反正都是状态机……
不管 C 语言还是汇编语言, 都是状态机.汇编语言的状态机太复杂, 可以把其简化成 C
语言. C 语言还太复杂, 我们还可以再简化: 我们只关心两个东西, 一个是纯粹的计算,
还有一个就是系统调用. 我们可以用一个更简化的语言.
我们真正关心的概念
- 应用程序 (高级语言状态机)
- 系统调用 (操作系统 API)
- 操作系统内部实现
没有人规定上面三者如何实现
- 通常的思路:真实的操作系统 + QEMU/NEMU 模拟器, 我们的思路
- 应用程序 = 纯粹计算的 Python 代码 + 系统调用
- 操作系统 = Python 系统调用实现,有 “假想” 的 I/O 设备
```py
def main():
sys_write('Hello, OS World')
```
== 操作系统 “玩具”:设计与实现
=== 操作系统玩具:API
四个 “系统调用” API
- `choose(xs)`: 返回 xs 中的一个随机选项
- `write(s)`: 输出字符串 s
- `spawn(fn)`: 创建一个可运行的状态机 fn
- `sched()`: 随机切换到任意状态机执行
除此之外,所有的代码都是确定 (deterministic) 的纯粹计算, 允许使用 list, dict
等数据结构
=== 操作系统玩具:应用程序
操作系统里面可以运行不止一个程序, 也就是 os 可以同时容纳好几个状态机.
所以最有意思的是`spawn(fn)`和`sched()`这两个系统调用.
操作系统玩具:我们可以动手把状态机画出来!
```py
count = 0
def Tprint(name):
global count
for i in range(3):
count += 1
sys_write(f'#{count:02} Hello from {name}{i+1}\n')
sys_sched()
def main():
n = sys_choose([3, 4, 5])
sys_write(f'#Thread = {n}\n')
for name in 'ABCDE'[:n]:
sys_spawn(Tprint, name)
sys_sched()
```
#tip("Tip")[
- 文件是操作系统的对象, 文件描述符就是一个指向操作系统对象的指针.
- 进程和线程也是操作系统的一个非常重要的对象, 即执行的状态机.线程共享内存, 进程有独立的内存.
]
状态机:
```
s0(ptr->{pc=.., n=3}, {Tp=1A},{Tp=1B},{Tp=1C})
--sched--> s0({pc=.., n=3}, {Tp=1A},ptr->{Tp=1B},{Tp=1C})
--write--> s0({pc=.., n=3}, {Tp=1A},ptr->{Tp=1B},{Tp=1C}, stdout='#1 Hello from B1')
```
```sh
❯ ./os-model.py threads.py
#Thread = 4
#01 Hello from C1
#02 Hello from A1
#03 Hello from A2
#04 Hello from D1
#05 Hello from B1
#06 Hello from B2
#07 Hello from B3
#08 Hello from C2
#09 Hello from A3
#10 Hello from D2
#11 Hello from C3
#12 Hello from D3
```
=== 实现系统调用
有些 “系统调用” 的实现是显而易见的
```py
def sys_write(s): print(s)
def sys_choose(xs): return random.choice(xs)
def sys_spawn(t): runnables.append(t)
```
有些就困难了
```py
def sys_sched():
raise NotImplementedError('No idea how')
```
#tip("Tip")[
`NotImplementedError`是个好的习惯.
]
我们需要
- 封存当前状态机的状态
- 恢复另一个 “被封存” 状态机的执行
- 没错,我们离真正的 “分时操作系统” 就只差这一步
==== ️🌶️ 借用 Python 的语言机制
Generator objects (无栈协程/轻量级线程/...)
```py
def numbers():
i = 0
while True:
ret = yield f'{i:b}' = “封存” 状态机状态
i += ret
```
使用方法:
```py
n = numbers() = 封存状态机初始状态
n.send(None) = 恢复封存的状态
n.send(0) = 恢复封存的状态 (并传入返回值)
```
完美适合我们实现操作系统玩具 (os-model.py)
=== 玩具的意义
我们并没有脱离真实的操作系统
- “简化” 了操作系统的 API
- 在暂时不要过度关注细节的时候理解操作系统
- 细节也会有的,但不是现在
- 学习路线:先 100% 理解玩具,再理解真实系统和玩具的差异
```c
void sys_write(const char *s) { printf("%s", s); }
void sys_sched() { usleep(rand() % 10000); }
int sys_choose(int x) { return rand() % x; }
void sys_spawn(void *(*fn)(void *), void *args) {
pthread_create(&threads[nthreads++], NULL, fn, args);
}
```
== 建模操作系统
=== 一个更 “全面” 的操作系统模型
进程 + 线程 + 终端 + 存储 (崩溃一致性)
| 系统调用/Linux 对应 | 行为 | | -------------------------------- |
------------------------------ | | `sys_spawn(fn)`/`pthread_create` | 创建从 fn
开始执行的线程 | | `sys_fork()`/`fork` | 创建当前状态机的完整复制 | | `sys_sched()`/定时被动调用
| 切换到随机的线程/进程执行 | | `sys_choose(xs)`/`rand` | 返回一个 xs
中的随机的选择 | | `sys_write(s)`/`printf` | 向调试终端输出字符串 s | | `sys_bread(k)`/`read` |
读取虚拟设磁盘块 k 的数据 | | `sys_bwrite(k, v)`/`write` | 向虚拟磁盘块 k
写入数据 v | | `sys_sync()`/`sync` | 将所有向虚拟磁盘的数据写入落盘 | | `sys_crash()`/长按电源按键
| 模拟系统崩溃 |
=== 模型做出的简化
- 被动进程/线程切换: 实际程序随时都可能被动调用 `sys_sched()` 切换
- 只有一个终端: 没有 `read()` (用 `choose` 替代 “允许读到任意值”)
- 磁盘是一个 dict:
- 把任意 key 映射到任意 value
- 实际的磁盘
- key 为整数
- value 是固定大小 (例如 4KB) 的数据
- 二者在某种程度上是可以 “互相转换” 的
=== 模型实现
原理与刚才的 “最小操作系统玩具” 类似
- #link("https://jyywiki.cn/pages/OS/2023/mosaic/mosaic.py")[ mosaic.py ] - 500
行建模操作系统
- 进程/线程都是 Generator Object
- 共享内存用 heap 变量访问
- 线程会得到共享 heap 的指针
- 进程会得到一个独立的 heap clone
输出程序运行的 “状态图”
- JSON Object
- Vertices: 线程/进程、内存快照、设备历史输出
- Edges: 系统调用
- 操作系统就是 “状态机的管理者”
=== 建模的意义
我们可以把状态机的执行画出来了!
- 可以直观地理解程序执行的全流程
- 可以对照程序在真实操作系统上的运行结果 这对于更复杂的程序来说是十分关键的
```c
void Tsum() {
for (int i = 0; i < n; i++) {
int tmp = sum;
tmp++;
// 假设此时可能发生进程/线程切换
sum = tmp;
}
}
```
假设10个线程, 每个线程都往sum上+10, sum的值最小可以是多少?
其中一个循环跑到最后一次循环然后读到一个1, tmp++变成2,
然后等其他线程都跑完再写入进去.故而最小值是 2.
== 编程实践
=== demo1
```py
def main():
x = sys_choose(['Head', 'Tail'])
x = x.lower()
sys_write('Hello, OS World')
```
#tip("Tip")[
为什么 `choose` 是系统调用, 因为不依靠外界, 是不可能靠纯粹的计算来实现真正的随机数的.
]
py 程序状态机:
```
s0
--choose--> s1(x='Head')
--cal--> s2(x='head')
--write--> s3(x='head)
```
发现对这个程序状态机来说, s3 和 s2 一样, 没有任何可观测的效果.
操作系统状态机的视角:
```
s0(stdout ='')
--choose--> s1(x='Head', stdout='')
--cal--> s2(x='head', stdout='')
--write--> s3(x='head', stdout='head')
```
操作系统里面可以运行不止一个程序, 也就是 os 可以同时容纳好几个状态机.
```py
def fn():
pass
def main():
= 创建了一个状态机
sys_spawn(fn)
```
操作系统状态机:
```
s0({ pc=main->1 })
--spawn--> s3({ pc=main->2 },{ pc=fn->1 })
```
问题: 下一步该执行哪个状态机?增加一个指针, 默认情况是不变的.但是`sched()`后会随机切换.
```
s0(ptr->{pc=main->1})
--spawn--> s3(ptr->{ pc=main->2 },{ pc=fn->1 })
--sched--> s3({ pc=main->2 }, ptr->{ pc=fn->1 })
```
=== python 的 generator
```py
❯ python3
Python 3.10.12 (main, Jun 11 2023, 05:26:28) [GCC 11.4.0] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>> def numbers():
... i = 0
... while True:
... ret = yield f'{i:b}' = “封存” 状态机状态
... i += ret
...
>>> n = numbers()
>>> type(n)
<class 'generator'>
>>> n.send(None)
'0'
>>> n.send(1)
'1'
>>> n.send(1)
'10'
>>> n.send(1)
'11'
>>> n.send(1)
'100'
>>> n.send(1)
'101'
>>> n.send(100)
'1101001'
>>> m = numbers()
>>> m.send(None)
'0'
>>> m.send(1)
'1'
>>> n.send(1)
'1101010'
```
#tip("Tip")[
generator 就是一个状态机. 一种是纯粹的计算, 一种是系统调用, 在 generator 里面就是 yield.`yield`可以把里面的信息暴露给外面, 然后把控制权交给外面.外面还可以把信息传回来.
]
#tip("Tip")[
怎么写一个装饰器?
]
=== C 语言版玩具
```c
static inline void
sys_sched() {
usleep(rand() % 10000);
}
// Constructor called before main()
static inline void __attribute__((constructor))
srand_init() {
srand(time(0));
}
// Destructor called after main()
static inline void __attribute__((destructor))
thread_join() {
for (int i = 0; i < nthreads; i++) {
pthread_join(threads[i], NULL); // Wait for thread terminations
}
}
```
为什么`sched`是随机睡眠?
这里的`constructor`和`destructor`是为了防止:
```c
int main(){
...
if(..){
return 1
}
release_res();
}
```
这种情况的资源泄露.
|
|
https://github.com/Area-53-Robotics/53E-Notebook-Over-Under-2023-2024 | https://raw.githubusercontent.com/Area-53-Robotics/53E-Notebook-Over-Under-2023-2024/giga-notebook/entries/notebook-toc.typ | typst | Creative Commons Attribution Share Alike 4.0 International | #import "/packages.typ": notebookinator
#import notebookinator: *
#import themes.radial.components: *
#show: create-body-entry.with(
title: "Typst Showcase: Table of Contents",
type: "notebook",
date: datetime(year: 2023, month: 7, day: 31),
author: "<NAME>",
witness: "<NAME>",
)
In past years our digital notebooks suffered from one major problem: the table
of contents was very difficult to maintain. If something in the introduction was
added, it would change every page number of every entry, which would then need
to be updated in the table of contents, for every entry, which was usually a
non-trivial amount of work, usually over an hour. We've solved this problem in
this notebook by automatically generating the table of contents.
The first thing we did was define a global variable to store all of our entries
in:
```typ
#let entries = state("entries", ())
```
Then we made a function that adds entries to this variable:
```typ
#let create_entry(title: "", type: "", start_date: none, end_date: none, body) = {
if start_date == none {
panic("No valid start date specified")
}
if end_date == none {
end_date = start_date
}
entries.update(x => {
x.push((
title: title,
type: type,
start_date: start_date,
end_date: end_date,
body: body,
))
x
})
}
```
Each entry is represented as a dictionary, which assigns key value pairs to all
of its relevant data, including title, type, start date, end date, and the
actual content of the entry.
Then we have a function that adds entries to the table of contents for every
entry in that global variable.
```typ
#let nb_toc() = {
page( // toc should be it's own page
footer: [#nb_frontmatter_footer()],
[
#heading(([Table of Contents])
#heading(([Entries], level: 1)
#locate(loc => {
for entry in entries.final(loc) { // creates a new row for each entry
[
#nb_label(label: entry.type)
#h(5pt)
#entry.title
#box(width: 1fr, line(length: 100%, stroke: (dash: "dotted")))
#entry.start_date.display("[year]/[month]/[day]") \
]
if not entry.end_date == entry.start_date {
align(right, [
#box(outset: 0pt, [
#line(
length: 15pt,
start: (-20pt, -8pt),
angle: 90deg,
stroke: (thickness: 1pt),
)
])
\
#entry.end_date.display("[year]/[month]/[day]")
])
}
}
})
#heading(([Appendix], level: 1)
Glossary \
#counter(page).update(_ => 0)
],
)
}
```
#v(100pt)
This means that all the notebooker has to do to add a new entry is this:
```typ
#nb_create_entry(
title: "Interesting Title",
type: "notebook"
start_date: datetime(year: 1970, month: 1, day: 1) // at the beginning of time
)[
// Content here
]
```
Which creates an entry in the table of contents that looks like this:
#let entry = (
title: "Interesting Title",
type: "notebook",
start_date: datetime(year: 1970, month: 1, day: 1),
)
#label(entry.type)
#h(5pt)
#entry.title
#box(width: 1fr, line(length: 100%, stroke: (dash: "dotted")))
#entry.start_date.display("[year]/[month]/[day]") \
|
https://github.com/WinstonMDP/knowledge | https://raw.githubusercontent.com/WinstonMDP/knowledge/master/complex_numbers.typ | typst | #import "cfg.typ": cfg
#show: cfg
= Комплексные числа
Поле комплексных чисел $CC$:
- $RR subset.eq CC$
- $i in CC: i^2 = -1$
- оно минимально
Поле комплексных чисел существует и единственно с точностью до изоморфизма.
Комплексное сопряжение:
$overline(a + b i) = a - b i$
$overline(alpha + beta) = overline(alpha) + overline(beta)$
$overline(alpha beta) = overline(alpha) overline(beta) $
$(a + b i) overline(a - b i) = a^2 + b^2$
$(a + b i)^(-1) = (a - b i)/(a^2 + b^2)$
$|a + b i| = sqrt(a^2 + b^2)$
|
|
https://github.com/jgm/typst-hs | https://raw.githubusercontent.com/jgm/typst-hs/main/test/typ/compiler/ops-invalid-08.typ | typst | Other | // Error: 3-12 cannot divide by zero
#(1.2 / 0.0)
|
https://github.com/vimkat/typst-ohm | https://raw.githubusercontent.com/vimkat/typst-ohm/main/src/lib.typ | typst | MIT License | // Colors
#import "lib/vars.typ": font, red, blue, yellow, light-blue, green, violet, dark-green
// Elements
#import "lib/elements.typ": pipe
// Components
#import "components/logo.typ": logo, logo-omega
// Templates
#import "templates/document.typ": document
#import "templates/event-sign.typ": event-sign
#import "templates/thesis.typ": thesis
#import "templates/presentation.typ"
|
https://github.com/simon-epfl/notes-ba3-simon | https://raw.githubusercontent.com/simon-epfl/notes-ba3-simon/main/comparch/notes.typ | typst | == Arithmetic/Logic instructions
"easy": two operands or one operand and a constant (immediate)
like `sll`, `add`, `xor`, `slt`, etc.
because immediate are limited in terms of space, we can use a register with `lui` (12 bits), then `addiu` (add unsigned) and `xor` to copy
== Assembler directives
Like `.text`, `.data`, `.asciiz`..
== Functions
`jal x1, sqrt` --> leaves `PC+4` into the `x1` register so the funtion can callback `x1` with a simple `jr x1` when finishing.
With RISCV we can simply use `jal offset` and `ret` instead of specifying the register `x1`.
#pagebreak()
== Stack Pointer
#image("sp_alloc.png")
#image("sp_inst.png")
#pagebreak()
== Passing Arguments
#image("passing_arg.png")
== Little/Big Endian
#image("little.png")
Little endian is used by RISCV and is cool because when using:
if `t0 = 1`:
```yasm
sw t0, 0(t1)
```
writes the same in memory as:
```yasm
sb t0, 0(t1)
```
!
|
|
https://github.com/seven-mile/blog-ng-content | https://raw.githubusercontent.com/seven-mile/blog-ng-content/master/_typst_ts_tmpl/physics.typ | typst | // Copyright 2023 Leedehai
// Use of this code is governed by a MIT license in the LICENSE.txt file.
// Current version: 0.7.3. Please see physics-manual.pdf for user docs.
// Returns whether a Content object holds an integer. The caller is responsible
// for ensuring the input argument is a Content object.
#let __content_holds_number(content) = {
return content.func() == text and regex("^\d+$") in content.text
}
// Given a Content generated from lr(), return the array of sub Content objects.
// Example: "[1,a_1,(1,1),n+1]" => "1", "a_1", "(1,1)", "n+1"
#let __extract_array_contents(content) = {
assert(type(content) == "content", message: "expecting a content type input")
if content.func() != math.lr { return none }
// A Content object made by lr() definitely has a "body" field, and a
// "children" field underneath it. It holds an array of Content objects,
// starting with a Content holding "(" and ending with a Content holding ")".
let children = content.at("body").at("children")
let result_elements = () // array of Content objects
// Skip the delimiters at the two ends.
let inner_children = children.slice(1, children.len() - 1)
// "a_1", "(1,1)" are all recognized as one AST node, respectively,
// because they are syntactically meaningful in Typst. However, things like
// "a+b", "a*b" are recognized as 3 nodes, respectively, because in Typst's
// view they are just plain sequences of symbols. We need to join the symbols.
let current_element_pieces = () // array of Content objects
for i in range(inner_children.len()) {
let e = inner_children.at(i)
if e == [ ] or e == [] { continue; }
if e != [,] { current_element_pieces.push(e) }
if e == [,] or (i == inner_children.len() - 1) {
if current_element_pieces.len() > 0 {
result_elements.push(current_element_pieces.join())
current_element_pieces = ()
}
continue;
}
}
return result_elements;
}
// A bare-minimum-effort symbolic addition.
#let __bare_minimum_effort_symbolic_add(elements) = {
assert(type(elements) == "array", message: "expecting an array of content")
let operands = () // array
for e in elements {
if not e.has("children") {
operands.push(e)
continue
}
// The elements is like "a+b" where there are multiple operands ("a", "b").
let current_operand = ()
let children = e.at("children")
for i in range(children.len()) {
let child = children.at(i)
if child == [+] {
operands.push(current_operand.join())
current_operand = ()
continue;
}
current_operand.push(child)
}
operands.push(current_operand.join())
}
let num_sum = 0
let map_id_to_sym = (:) // dictionary, symbol repr to symbol
let map_id_to_sym_sum = (:) // dictionary, symbol repr to number
for e in operands {
if __content_holds_number(e) {
num_sum += int(e.text)
continue
}
let is_num_times_sth = (
e.has("children") and __content_holds_number(e.at("children").at(0)))
if is_num_times_sth {
let leading_num = int(e.at("children").at(0).text)
let sym = e.at("children").slice(1).join() // join to one symbol
let sym_id = repr(sym) // string
if sym_id in map_id_to_sym {
let sym_sum_so_far = map_id_to_sym_sum.at(sym_id) // number
map_id_to_sym_sum.insert(sym_id, sym_sum_so_far + leading_num)
} else {
map_id_to_sym.insert(sym_id, sym)
map_id_to_sym_sum.insert(sym_id, leading_num)
}
} else {
let sym = e
let sym_id = repr(sym) // string
if repr(e) in map_id_to_sym {
let sym_sum_so_far = map_id_to_sym_sum.at(sym_id) // number
map_id_to_sym_sum.insert(sym_id, sym_sum_so_far + 1)
} else {
map_id_to_sym.insert(sym_id, sym)
map_id_to_sym_sum.insert(sym_id, 1)
}
}
}
let expr_terms = () // array of Content object
let sorted_sym_ids = map_id_to_sym.keys().sorted()
for sym_id in sorted_sym_ids {
let sym = map_id_to_sym.at(sym_id)
let sym_sum = map_id_to_sym_sum.at(sym_id) // number
if sym_sum == 1 {
expr_terms.push(sym)
} else if sym_sum != 0 {
expr_terms.push([#sym_sum #sym])
}
}
if num_sum != 0 {
expr_terms.push([#num_sum]) // make a Content object holding the number
}
return expr_terms.join([+])
}
// == Braces
#let Set(..sink) = style(styles => {
let args = sink.pos() // array
let expr = args.at(0, default: none)
let cond = args.at(1, default: none)
let height = measure($ expr cond $, styles).height;
let phantom = box(height: height, width: 0pt, inset: 0pt, stroke: none);
if expr == none {
if cond == none { ${}$ } else { ${lr(|phantom#h(0pt))#cond}$ }
} else {
if cond == none { ${#expr}$ } else { ${#expr lr(|phantom#h(0pt))#cond}$ }
}
})
#let order(content) = $cal(O)(content)$
#let evaluated(content) = {
$lr(zwj#content|)$
}
#let eval = evaluated
#let expectationvalue(f) = $lr(angle.l #f angle.r)$
#let expval = expectationvalue
// == Vector notations
#let vecrow(..content) = $lr(( #content.pos().join(",") ))$
#let TT = $sans(upright(T))$
#let vectorbold(a) = $bold(italic(#a))$
#let vb = vectorbold
#let __vectoraccent(a, accent) = {
let bold_italic(e) = math.bold(math.italic(e))
if type(a) == "content" and a.func() == math.attach {
math.attach(
math.accent(bold_italic(a.base), accent),
t: if a.has("t") { math.bold(a.t) } else { none },
b: if a.has("b") { math.bold(a.b) } else { none },
tl: if a.has("tl") { math.bold(a.tl) } else { none },
bl: if a.has("bl") { math.bold(a.bl) } else { none },
tr: if a.has("tr") { math.bold(a.tr) } else { none },
br: if a.has("br") { math.bold(a.br) } else { none },
)
} else {
math.accent(bold_italic(a), accent)
}
}
#let vectorarrow(a) = __vectoraccent(a, math.arrow)
#let va = vectorarrow
#let vectorunit(a) = __vectoraccent(a, math.hat)
#let vu = vectorunit
#let gradient = $bold(nabla)$
#let grad = gradient
#let divergence = $bold(nabla)dot.c$
#let div = divergence
#let curl = $bold(nabla)times$
#let laplacian = $nabla^2$
#let dotproduct = $dot$
#let dprod = dotproduct
#let crossproduct = $times$
#let cprod = crossproduct
// == Matrices
#let matrixdet(..sink) = {
math.mat(..sink, delim:"|")
}
#let mdet = matrixdet
#let diagonalmatrix(..sink) = {
let (args, kwargs) = (sink.pos(), sink.named()) // array, dictionary
let delim = kwargs.at("delim", default:"(")
let fill = kwargs.at("fill", default: none)
let arrays = () // array of arrays
let n = args.len()
for i in range(n) {
let array = range(n).map((j) => {
let e = if j == i { args.at(i) } else { fill }
return e
})
arrays.push(array)
}
math.mat(delim: delim, ..arrays)
}
#let dmat = diagonalmatrix
#let antidiagonalmatrix(..sink) = {
let (args, kwargs) = (sink.pos(), sink.named()) // array, dictionary
let delim = kwargs.at("delim", default:"(")
let fill = kwargs.at("fill", default: none)
let arrays = () // array of arrays
let n = args.len()
for i in range(n) {
let array = range(n).map((j) => {
let complement = n - 1 - i
let e = if j == complement { args.at(i) } else { fill }
return e
})
arrays.push(array)
}
math.mat(delim: delim, ..arrays)
}
#let admat = antidiagonalmatrix
#let identitymatrix(order, delim:"(", fill:none) = {
let order_num = 1
if type(order) == "content" and __content_holds_number(order) {
order_num = int(order.text)
} else {
panic("the order shall be an integer, e.g. 2")
}
let ones = range(order_num).map((i) => 1)
diagonalmatrix(..ones, delim: delim, fill: fill)
}
#let imat = identitymatrix
#let zeromatrix(order, delim:"(") = {
let order_num = 1
if type(order) == "content" and __content_holds_number(order) {
order_num = int(order.text)
} else {
panic("the order shall be an integer, e.g. 2")
}
let ones = range(order_num).map((i) => 0)
diagonalmatrix(..ones, delim: delim, fill: 0)
}
#let zmat = zeromatrix
#let jacobianmatrix(fs, xs, delim:"(") = {
assert(type(fs) == "array", message: "expecting an array of function names")
assert(type(xs) == "array", message: "expecting an array of variable names")
let arrays = () // array of arrays
for f in fs {
arrays.push(xs.map((x) => math.frac($diff#f$, $diff#x$)))
}
math.mat(delim: delim, ..arrays)
}
#let jmat = jacobianmatrix
#let hessianmatrix(fs, xs, delim:"(") = {
assert(type(fs) == "array", message: "expecting a one-element array")
assert(fs.len() == 1, message: "expecting only one function name")
let f = fs.at(0)
assert(type(xs) == "array", message: "expecting an array of variable names")
let row_arrays = () // array of arrays
let order = xs.len()
for r in range(order) {
let row_array = () // array
let xr = xs.at(r)
for c in range(order) {
let xc = xs.at(c)
row_array.push(math.frac(
$diff^#order #f$,
if xr == xc { $diff #xr^2$ } else { $diff #xr diff #xc$ }
))
}
row_arrays.push(row_array)
}
math.mat(delim: delim, ..row_arrays)
}
#let hmat = hessianmatrix
#let xmatrix(m, n, func, delim:"(") = {
let rows = none
if type(m) == "content" and __content_holds_number(m) {
rows = int(m.text)
} else {
panic("the first argument shall be an integer, e.g. 2")
}
let cols = none
if type(n) == "content" and __content_holds_number(m) {
cols = int(n.text)
} else {
panic("the second argument shall be an integer, e.g. 2")
}
assert(
type(func) == "function",
message: "func shall be a function (did you forget to add a preceding '#' before the function name)?"
)
let row_arrays = () // array of arrays
for i in range(1, rows + 1) {
let row_array = () // array
for j in range(1, cols + 1) {
row_array.push(func(i, j))
}
row_arrays.push(row_array)
}
math.mat(delim: delim, ..row_arrays)
}
#let xmat = xmatrix
// == Dirac braket notations
#let bra(f) = $lr(angle.l #f|)$
#let ket(f) = $lr(|#f angle.r)$
// Credit: thanks to peng1999@ and szdytom@'s suggestions of measure() and
// phantoms. The hack works until https://github.com/typst/typst/issues/240 is
// addressed by Typst.
#let braket(..sink) = style(styles => {
let args = sink.pos() // array
assert(args.len() == 1 or args.len() == 2, message: "expecting 1 or 2 args")
let bra = args.at(0)
let ket = args.at(1, default: bra)
let height = measure($ bra ket $, styles).height;
let phantom = box(height: height, width: 0pt, inset: 0pt, stroke: none);
$ lr(angle.l bra lr(|phantom#h(0pt)) ket angle.r) $
})
// Credit: until https://github.com/typst/typst/issues/240 is addressed by Typst
// we use the same hack as braket().
#let ketbra(..sink) = style(styles => {
let args = sink.pos() // array
assert(args.len() == 1 or args.len() == 2, message: "expecting 1 or 2 args")
let bra = args.at(0)
let ket = args.at(1, default: bra)
let height = measure($ bra ket $, styles).height;
let phantom = box(height: height, width: 0pt, inset: 0pt, stroke: none);
$ lr(|bra#h(0pt)phantom angle.r)lr(angle.l phantom#h(0pt)ket|) $
})
#let innerproduct = braket
#let iprod = innerproduct
#let outerproduct = ketbra
#let oprod = outerproduct
// Credit: until https://github.com/typst/typst/issues/240 is addressed by Typst
// we use the same hack as braket().
#let matrixelement(n, M, m) = style(styles => {
let height = measure($ #n #M #m $, styles).height;
let phantom = box(height: height, width: 0pt, inset: 0pt, stroke: none);
$ lr(angle.l #n |#M#h(0pt)phantom| #m angle.r) $
})
#let mel = matrixelement
// == Math functions
#let sin = math.op("sin")
#let sinh = math.op("sinh")
#let arcsin = math.op("arcsin")
#let asin = math.op("asin")
#let cos = math.op("cos")
#let cosh = math.op("cosh")
#let arccos = math.op("arccos")
#let acos = math.op("acos")
#let tan = math.op("tan")
#let tanh = math.op("tanh")
#let arctan = math.op("arctan")
#let atan = math.op("atan")
#let sec = math.op("sec")
#let sech = math.op("sech")
#let arcsec = math.op("arcsec")
#let asec = math.op("asec")
#let csc = math.op("csc")
#let csch = math.op("csch")
#let arccsc = math.op("arccsc")
#let acsc = math.op("acsc")
#let cot = math.op("cot")
#let coth = math.op("coth")
#let arccot = math.op("arccot")
#let acot = math.op("acot")
#let diag = math.op("diag")
#let trace = math.op("trace")
#let tr = math.op("tr")
#let Trace = math.op("Trace")
#let Tr = math.op("Tr")
#let rank = math.op("rank")
#let erf = math.op("erf")
#let Res = math.op("Res")
#let Re = math.op("Re")
#let Im = math.op("Im")
#let sgn = $op("sgn")$
// == Differentials
#let differential(..sink) = {
let (args, kwargs) = (sink.pos(), sink.named()) // array, dictionary
let orders = ()
let var_num = args.len()
let default_order = [1] // a Content holding "1"
let last = args.at(args.len() - 1)
if type(last) == "content" {
if last.func() == math.lr and last.at("body").at("children").at(0) == [\[] {
var_num -= 1
orders = __extract_array_contents(last) // array
} else if __content_holds_number(last) {
var_num -= 1
default_order = last // treat as a single element
orders.push(default_order)
}
} else if type(last) == "integer" {
var_num -= 1
default_order = [#last] // make it a Content
orders.push(default_order)
}
let dsym = kwargs.at("d", default: $upright(d)$)
let prod = kwargs.at("p", default: none)
let difference = var_num - orders.len()
while difference > 0 {
orders.push(default_order)
difference -= 1
}
let arr = ()
for i in range(var_num) {
let (var, order) = (args.at(i), orders.at(i))
if order != [1] {
arr.push($dsym^#order#var$)
} else {
arr.push($dsym#var$)
}
}
$#arr.join(prod)$
}
#let dd = differential
#let variation = dd.with(d: sym.delta)
#let var = variation
// Do not name it "delta", because it will collide with "delta" in math
// expressions (note in math mode "sym.delta" can be written as "delta").
#let difference = dd.with(d: sym.Delta)
#let __combine_var_order(var, order) = {
let naive_result = math.attach(var, t: order)
if type(var) != "content" or var.func() != math.attach {
return naive_result
}
if var.has("b") and (not var.has("t")) {
// Place the order superscript directly above the subscript, as is
// the custom is most papers.
return math.attach(var.base, t: order, b: var.b)
}
// Even if var.has("t") is true, we don't take any special action. Let
// user decide. Say, if they want to wrap var in a "(..)", let they do it.
return naive_result
}
#let derivative(f, ..sink) = {
if f == [] { f = none } // Convert empty content to none
let (args, kwargs) = (sink.pos(), sink.named()) // array, dictionary
assert(args.len() > 0, message: "variable name expected")
let d = kwargs.at("d", default: $upright(d)$)
let slash = kwargs.at("s", default: none)
let var = args.at(0)
assert(args.len() >= 1, message: "expecting at least one argument")
let display(num, denom, slash) = {
if slash == none {
$#num/#denom$
} else {
let sep = (sym.zwj, slash, sym.zwj).join()
$#num#sep#denom$
}
}
if args.len() >= 2 { // i.e. specified the order
let order = args.at(1) // Not necessarily representing a number
let upper = if f == none { $#d^#order$ } else { $#d^#order#f$ }
let varorder = __combine_var_order(var, order)
display(upper, $#d#varorder$, slash)
} else { // i.e. no order specified
let upper = if f == none { $#d$ } else { $#d#f$ }
display(upper, $#d#var$, slash)
}
}
#let dv = derivative
#let partialderivative(..sink) = {
let (args, kwargs) = (sink.pos(), sink.named()) // array, dictionary
assert(args.len() >= 2, message: "expecting one function name and at least one variable name")
let f = args.at(0)
if f == [] { f = none } // Convert empty content to none
let var_num = args.len() - 1
let orders = ()
let default_order = [1] // a Content holding "1"
// The last argument might be the order numbers, let's check.
let last = args.at(args.len() - 1)
if type(last) == "content" {
if last.func() == math.lr and last.at("body").at("children").at(0) == [\[] {
var_num -= 1
orders = __extract_array_contents(last) // array
} else if __content_holds_number(last) {
var_num -= 1
default_order = last
orders.push(default_order)
}
} else if type(last) == "integer" {
var_num -= 1
default_order = [#last] // make it a Content
orders.push(default_order)
}
let difference = var_num - orders.len()
while difference > 0 {
orders.push(default_order)
difference -= 1
}
let total_order = none // any type, could be a number
// Do not use kwargs.at("total", default: ...), so as to avoid unnecessary
// premature evaluation of the default param.
total_order = if "total" in kwargs {
kwargs.at("total")
} else {
__bare_minimum_effort_symbolic_add(orders)
}
let lowers = ()
for i in range(var_num) {
let var = args.at(1 + i) // 1st element is the function name, skip
let order = orders.at(i)
if order == [1] {
lowers.push($diff#var$)
} else {
let varorder = __combine_var_order(var, order)
lowers.push($diff#varorder$)
}
}
let upper = if total_order != 1 and total_order != [1] { // number or Content
if f == none { $diff^#total_order$ } else { $diff^#total_order#f$ }
} else {
if f == none { $diff$ } else { $diff #f$ }
}
let display(num, denom, slash) = {
if slash == none {
math.frac(num, denom)
} else {
let sep = (sym.zwj, slash, sym.zwj).join()
$#num#sep#denom$
}
}
let slash = kwargs.at("s", default: none)
display(upper, lowers.join(), slash)
}
#let pdv = partialderivative
// == Miscellaneous
// With the default font, the original symbol `planck.reduce` has a slash on the
// letter "h", and it is different from the usual "hbar" symbol, which has a
// horizontal bar on the letter "h".
//
// Here, we manually create a "hbar" symbol by adding the font-independent
// horizontal bar produced by strike() to the current font's Planck symbol, so
// that the new "hbar" symbol and the existing Planck symbol look similar in any
// font (not just "New Computer Modern").
//
// However, strike() causes some side effects in math mode: it shifts the symbol
// downward. This seems like a Typst bug. Therefore, we need to use move() to
// eliminate those side effects so that the symbol behave nicely in math
// expressions.
//
// We also need to use wj (word joiner) to eliminate the unwanted horizontal
// spaces that manifests when using the symbol in math mode.
//
// Credit: Enivex in https://github.com/typst/typst/issues/355 was very helpful.
#let hbar = (sym.wj, move(dy: -0.08em, strike(offset: -0.55em, extent: -0.05em, sym.planck)), sym.wj).join()
#let tensor(T, ..sink) = {
let args = sink.pos()
let (uppers, lowers) = ((), ()) // array, array
let hphantom(s) = { hide(box(height: 0em, s)) } // Like Latex's \hphantom
for i in range(args.len()) {
let arg = args.at(i)
let tuple = if arg.has("children") {
arg.at("children")
} else {
([+], sym.square)
}
assert(type(tuple) == "array", message: "shall be array")
let pos = tuple.at(0)
let symbol = if tuple.len() >= 2 {
tuple.slice(1).join()
} else {
sym.square
}
if pos == [+] {
let rendering = $#symbol$
uppers.push(rendering)
lowers.push(hphantom(rendering))
} else { // Curiously, equality with [-] is always false, so we don't do it
let rendering = $#symbol$
uppers.push(hphantom(rendering))
lowers.push(rendering)
}
}
// Do not use "...^..._...", because the lower indices appear to be placed
// slightly lower than a normal subscript.
// Use a phantom with zwj (zero-width word joiner) to vertically align the
// starting points of the upper and lower indices. Also, we put T inside
// the first argument of attach(), so that the indices' vertical position
// auto-adjusts with T's height.
math.attach((T,hphantom(sym.zwj)).join(), t: uppers.join(), b: lowers.join())
}
#let isotope(element, /*atomic mass*/a: none, /*atomic number*/z: none) = {
$attach(upright(element), tl: #a, bl: #z)$
}
#let __signal_element(e, W, color) = {
let style = 0.5pt + color
if e == "&" {
return rect(width: W, height: 1em, stroke: none)
} else if e == "n" {
return rect(width: 1em, height: W, stroke: (left: style, top: style, right: style))
} else if e == "u" {
return rect(width: W, height: 1em, stroke: (left: style, bottom: style, right: style))
} else if (e == "H" or e == "1") {
return rect(width: W, height: 1em, stroke: (top: style))
} else if e == "h" {
return rect(width: W * 50%, height: 1em, stroke: (top: style))
} else if e == "^" {
return rect(width: W * 10%, height: 1em, stroke: (top: style))
} else if (e == "M" or e == "-") {
return line(start: (0em, 0.5em), end: (W, 0.5em), stroke: style)
} else if e == "m" {
return line(start: (0em, 0.5em), end: (W * 0.5, 0.5em), stroke: style)
} else if (e == "L" or e == "0") {
return rect(width: W, height: 1em, stroke: (bottom: style))
} else if e == "l" {
return rect(width: W * 50%, height: 1em, stroke: (bottom: style))
} else if e == "v" {
return rect(width: W * 10%, height: 1em, stroke: (bottom: style))
} else if e == "=" {
return rect(width: W, height: 1em, stroke: (top: style, bottom: style))
} else if e == "#" {
return path(stroke: style, closed: false,
(0em, 0em), (W * 50%, 0em), (0em, 1em), (W, 1em),
(W * 50%, 1em), (W, 0em), (W * 50%, 0em),
)
} else if e == "|" {
return line(start: (0em, 0em), end: (0em, 1em), stroke: style)
} else if e == "'" {
return line(start: (0em, 0em), end: (0em, 0.5em), stroke: style)
} else if e == "," {
return line(start: (0em, 0.5em), end: (0em, 1em), stroke: style)
} else if e == "R" {
return line(start: (0em, 1em), end: (W, 0em), stroke: style)
} else if e == "F" {
return line(start: (0em, 0em), end: (W, 1em), stroke: style)
} else if e == "<" {
return path(stroke: style, closed: false, (W, 0em), (0em, 0.5em), (W, 1em))
} else if e == ">" {
return path(stroke: style, closed: false, (0em, 0em), (W, 0.5em), (0em, 1em))
} else if e == "C" {
return path(stroke: style, closed: false, (0em, 1em), ((W, 0em), (-W * 75%, 0.05em)))
} else if e == "c" {
return path(stroke: style, closed: false, (0em, 1em), ((W * 50%, 0em), (-W * 38%, 0.05em)))
} else if e == "D" {
return path(stroke: style, closed: false, (0em, 0em), ((W, 1em), (-W * 75%, -0.05em)))
} else if e == "d" {
return path(stroke: style, closed: false, (0em, 0em), ((W * 50%, 1em), (-W * 38%, -0.05em)))
} else if e == "X" {
return path(stroke: style, closed: false,
(0em, 0em), (W * 50%, 0.5em), (0em, 1em),
(W, 0em), (W * 50%, 0.5em), (W, 1em),
)
} else {
return "[" + e + "]"
}
}
#let signals(str, step: 1em, color: black) = {
assert(type(str) == "string", message: "input needs to be a string")
let elements = () // array
let previous = " "
for e in str {
if e == " " { continue; }
if e == "." {
elements.push(__signal_element(previous, step, color))
} else {
elements.push(__signal_element(e, step, color))
previous = e
}
}
grid(
columns: (auto,) * elements.len(),
column-gutter: 0em,
..elements,
)
}
#let BMEsymadd(content) = {
let elements = __extract_array_contents(content)
__bare_minimum_effort_symbolic_add(elements)
}
// Add symbol definitions to the corresponding sections. Do not simply append
// them at the end of file.
|
|
https://github.com/RaphGL/ElectronicsFromBasics | https://raw.githubusercontent.com/RaphGL/ElectronicsFromBasics/main/DC/chap4/2_arithmetic_with_scientific_notation.typ | typst | Other | #import "../../core/core.typ"
=== Arithmetic with scientific notation
The benefits of scientific notation do not end with ease of writing and
expression of accuracy. Such notation also lends itself well to
mathematical problems of multiplication and division. Let\'s say we
wanted to know how many electrons would flow past a point in a circuit
carrying 1 amp of electric current in 25 seconds. If we know the number
of electrons per second in the circuit (which we do), then all we need
to do is multiply that quantity by the number of seconds (25) to arrive
at an answer of total electrons:
$ 6,250,000,000,000,000,000 "electrons per second" times 25 "seconds" = \
156,250,000,000,000,000,000 "electrons passing by in 25 seconds" $
Using scientific notation, we can write the problem like this:
$ 6.25 times 10^18 "electrons per second" times 25 "seconds" $
If we take the \"6.25\" and multiply it by 25, we get 156.25. So, the
answer could be written as:
$ 156.25 times 10^18 "electrons" $
However, if we want to hold to standard convention for scientific
notation, we must represent the significant digits as a number between 1
and 10. In this case, we\'d say \"1.5625\" multiplied by some
power-of-ten. To obtain 1.5625 from 156.25, we have to skip the decimal
point two places to the left. To compensate for this without changing
the value of the number, we have to raise our power by two notches (10
to the 20th power instead of 10 to the 18th):
$ 1.5625 times 10^20 "electrons" $
What if we wanted to see how many electrons would pass by in 3,600
seconds (1 hour)? To make our job easier, we could put the time in
scientific notation as well:
$ (6.25 times 10^18 "electrons per second") times (3.6 times 10^3 "seconds") $
To multiply, we must take the two significant sets of digits (6.25 and
3.6) and multiply them together; and we need to take the two
powers-of-ten and multiply them together. Taking 6.25 times 3.6, we get
22.5. Taking $10^18$ times $10^3$, we get $10^21$
(exponents with common base numbers add). So, the answer is:
$
22.5 times 10^21 "electrons"
"... or more properly ..."
2.25 times 10^22 "electrons"
$
To illustrate how division works with scientific notation, we could
figure that last problem \"backwards\" to find out how long it would
take for that many electrons to pass by at a current of 1 amp:
$ (2.25 times 10^22 "electrons") / (6.25 times 10^18 "electrons per second") $
Just as in multiplication, we can handle the significant digits and
powers-of-ten in separate steps (remember that you subtract the
exponents of divided powers-of-ten):
$ (2.25 / 6.25) times (10^22 / 10^18) $
And the answer is: $0.36 times 10^4$, or $3.6 times 10^3$, seconds.
You can see that we arrived at the same quantity of time (3600 seconds).
Now, you may be wondering what the point of all this is when we have
electronic calculators that can handle the math automatically. Well,
back in the days of scientists and engineers using \"slide rule\" analog
computers, these techniques were indispensable. The \"hard\" arithmetic
(dealing with the significant digit figures) would be performed with the
slide rule while the powers-of-ten could be figured without any help at
all, being nothing more than simple addition and subtraction.
#core.review[
- Significant digits are representative of the real-world accuracy of a
number.
- Scientific notation is a \"shorthand\" method to represent very large
and very small numbers in easily-handled form.
- When multiplying two numbers in scientific notation, you can multiply
the two significant digit figures and arrive at a power-of-ten by
adding exponents.
- When dividing two numbers in scientific notation, you can divide the
two significant digit figures and arrive at a power-of-ten by
subtracting exponents.
]
|
https://github.com/typst/packages | https://raw.githubusercontent.com/typst/packages/main/packages/preview/cetz/0.3.0/src/version.typ | typst | Apache License 2.0 | #let version = version(0,3,0)
|
https://github.com/Area-53-Robotics/53E-Notebook-Over-Under-2023-2024 | https://raw.githubusercontent.com/Area-53-Robotics/53E-Notebook-Over-Under-2023-2024/giga-notebook/entries/intake-rebuild/identify.typ | typst | Creative Commons Attribution Share Alike 4.0 International | #import "/packages.typ": notebookinator
#import notebookinator: *
#import themes.radial.components: *
#show: create-body-entry.with(
title: "Identify: Intake Rebuild",
type: "identify",
date: datetime(year: 2024, month: 2, day: 10), // TODO: fix date
author: "<NAME>",
witness: "<NAME>",
)
At our past tournaments we began to see some problems with our intake. Poor
design was causing it to fall apart in several ways. The complete lack of
bracing meant that the c-channel was bending, the motor was exposed to the
outside of the robot, meaning it could easily be hit and disassembled by other
robots, and the stops weren't boxed at all, meaning they were twisting the
c-channel they were screwed into.
Our intake is our main method of scoring this late in the season, so its
extremely important that it be working as well as possible.
#image("./old-intake.png")
= Constraints
- We can only use 1 motor for this design.
- The design must also fit within the starting 18", and cannot expand further than
36". This is particularly a challenge for the intake because we need it to stick
out of the robot in order to grab the triballs easily.
= Goals
- Protect the motor powering the intake by placing it on the inside of the bot.
- Brace the intake to remove pressure from the axle.
- Make the intake wider to make possession easier.
- Be able to possess triballs at the same rate as the old intake.
|
https://github.com/yonatankremer/matrix-utils | https://raw.githubusercontent.com/yonatankremer/matrix-utils/main/test.typ | typst | MIT License | #import "src/complex.typ": *
#import "src/matrix2.typ": *
//#madd($mat(1,2;3,5)$,$mat(1,2;3,4)$,)
//#mrow-switch($mat(1,2;3,5)$,0,1)
//#mrow-mul($mat(1,2;3,5)$,0,2)
//#mrow-add($mat(1,2;3,5)$,0,1,1)
#let c = cadd(1,2)
#_cunform(c) |
https://github.com/YDX-2147483647/statistical-dsp | https://raw.githubusercontent.com/YDX-2147483647/statistical-dsp/main/report.typ | typst | #import "@preview/physica:0.8.1": pdv, order
#import "template.typ": project
#show: project.with(
title: "Experimental and Simulation Training",
author: yaml("assets/author.yaml"),
date: "2023-11 – 2023-12",
info: (
Course: [Statistical Digital Signal Processing],
Teacher: [杨小鹏、曾小路],
"Student name": "{name}",
"Student ID": "{id}",
"Class ID": "{class_id}",
School: [Information and Electronics],
Major: [Electronic Engineering],
"Laboratory time": "{date}",
Location: [(Known but random)],
"Experiment type": [Principle verification],
Partner: [(None)],
Score: none,
),
)
#let expect = math.op("𝔼")
#let variant = math.op("𝕍")
= Sinusoidal frequency estimation and Cramér--Rao lower bound
== Cramér--Rao lower bound
The support of likelihood $p$ is always $RR$, so does not depend on the parameter $f_0$. Moreover, PDF is smooth, hence $pdv(,theta) p$ and $pdv(,theta) integral p dif x$ exist everywhere. As a result, the problem is regular and Cramér--Rao lower bound (CRLB) holds.
The average log likelihood
$
(ln p) / N
= - ln sqrt(2pi sigma^2) - overline((x[n] - s[n;f_0])^2) / (2 sigma^2),
$
where
$
s[n; f_0] = A cos(2pi f_0 n + phi.alt)
$
and
$
pdv(,f_0) s[n; f_0] &= -2 pi n A sin(2pi f_0 n + phi.alt). \
// pdv(,f_0, 2) s[n; f_0] &= -(2 pi n)^2 s[n; f_0]. \
$
We can derive that
$
pdv(,f_0) (x - s)^2 / 2 &= -(x - s) pdv(,f_0) s. \
pdv(,f_0, 2) (x - s)^2 / 2
&= -(x - s) pdv(,f_0,2) s + (pdv(,f_0) s)^2. \
// &= (x - s) (2 pi n)^2 s + (pdv(,f_0) s)^2 \
// &= (2 pi n)^2 x s + (2pi n A)^2 (sin^2(2pi f_0 n + phi.alt) - cos^2(2pi f_0 n + phi.alt)) \
// &= (2 pi n)^2 x s - (2pi n A)^2 cos(4pi f_0 n + phi.alt). \
$
Therefore, the average observed Fisher information
$
- pdv(,f_0, 2) (ln p) / N
&= 1/sigma^2 overline(pdv(,f_0, 2) (x - s)^2 / 2) \
&= - 1/sigma^2 overline((x - s) pdv(,f_0,2) s - (pdv(,f_0) s)^2). \
$
Take expectation and substitute $expect x = s$, and we get Fisher information
$
-N expect pdv(,f_0, 2) (ln p) / N
&= N/sigma^2 overline((pdv(,f_0) s)^2) \
&:= 1/sigma^2 sum_n (pdv(,f_0) s[n; f_0])^2 \
&= ((2pi A) / sigma)^2 sum_n n^2 sin^2(2pi f_0 n + phi.alt). \
$
CRLB is the inverse of that, namely
$
(sigma / (2pi A))^2 1 / (sum_n n^2 sin^2(2pi f_0 n + phi.alt)).
$
If we approximate all $sin^2 (dots.c)$ as
$1/(2pi) integral_0^(2pi) sin^2 theta dif theta = 1/2$,
then we know CRLB is about
$
(sigma / (2pi A))^2 1 / (sum_n 1/2 n^2)
&approx (sigma / (2pi A))^2 6/(N (N-1/2) (N-1)) \
&= order(1 / N^3).
$
== Plot
#figure(
image("fig/CRLB.png", width: 80%),
caption: [
Cramér--Rao lower bound for sinusoidal frequency estimation
$A^2 = sigma^2$, $N = 10$, $phi.alt = 0$.
]
)
- The bound is *symmetric* about $f_0 = 1/4$.
$sin(2pi (1/2 - f_0) n) = (-1)^n sin(2pi f_0 n)$. We do not care about “$plus.minus$”, so the bound are same for $f_0$ and $1/2 - f_0$.
- The *approximation* of $sin^2(dots.c) approx 1/2$ holds, and it is more accurate for middle frequencies ($f_0 approx 1/4$).
For middle frequencies, the phase looks more random (not just $0$ or $pi$), so $sum sin^2(dots.c)$ becomes similar to $integral sin^2 theta dif theta$. That is, errors of the approximation get canceled out more easily.
- The bound *oscillates* across $f_0$, and there are *preferred frequencies* ($f_0$ with smaller bound) around $f_0 approx 1/(2N), 2/(2N), ..., (N-1)/(2N)$.
$sin(2pi f_0 n)$ takes different values for different $f_0$. Its relative amplitude to noise, $sin(dots.c) / sigma$, gives us information about $f_0$ --- Fisher information varies with $f_0$, so CRLB also varies.
If $f_0 approx m/(2N)$, where $m = 1,...,N-1$, then $sin(2pi f_0 n) approx sin(pi/N m n)$ will roughly attain $plus.minus 1$ for some $n$, so we are able to estimate more accurately.
- The bound *goes to $+oo$* as $f_0 -> 0^+$ or $f_0 -> (1/2)^-$.
$sin(0 n) equiv 0$ ($f_0 = 0$) and $sin(pi n) equiv 0$ ($f_0 = 1/2$). Thus, a slight change in frequency will not alter the signal significantly, making it hard to estimate.
= Sinusoidal amplitude estimation and best linear unbiased estimator
== Estimate the amplitude
We know first two moments of the distribution, but we have no knowledge of PDF (probability density function). Therefore, we prefer best linear unbiased estimator (BLUE).
Observation matrix
$
H = mat(
cos(2pi f_1 times 0);
cos(2pi f_1 times 1);
dots.v;
cos(2pi f_1 (N-1));
).
$
Covariance
$
C_(i j) = cases(
1.81 &space i = j,
0.9 &space i = j plus.minus 2,
0 &space "other",
), quad i,j in {1,...,N}.
$
Then BLUE is
$
hat(A) = (H^dagger C^(-1) H)^(-1) H^dagger C^(-1) mat(x[0]; x[1]; dots.v; x[N-1]),
$
where $H^dagger$ means $H$ transposed.
== Power spectral density
Power spectral density (PSD) of $w$ is the discrete time Fourier transform of its auto-correlation function (ACF):
$
P_w (f)
&= 1.81 e^(2pi i f times 0) + 0.9 e^(2pi i f times 2) + 0.9 e^(2pi i f times (-2)) \
&= 1.81 + 1.8 cos(4pi f). \
$
#figure(
image("fig/PSD.png", width: 60%),
caption: [PSD of $w$]
) <fig:PSD>
- The auto-correlation is real and *symmetric about $0$* (and conjugate symmetric about $0$), so is the PSD.
That is why we only plot for $f in [0,1/2]$.
- PSD is *symmetric about $1/4$*.
Knowing PSD is symmetric about $0$, this is equivalent to period $1/2$.
The reason is that ACF only has nonzero values at even $k$, and $e^(j 2pi times 1/2 times 2) = 1$.
- PSD is *high at $0$ and $1/2$*.
Because ACF has positive total energy, and PSD has period $1/2$ as mentioned above.
- PSD is *low at $1/4$*.
Frequency $1/4$ corresponds to period $4$, or $+1, 0, -1, 0$ in ACF. But the actual ACF is $++, 0, +, 0$, which does not match perfectly with $+,0, -, 0$. Therefore PSD is low at $1/4$.
_Remarks._
The document did not specify whose PSD should be of concerned. PSD of $w$ is strongly related to performance of $hat(A)$, so I discuss it here. Besides, PSD of $x = A cos(2pi f_1 n) + w$ is $P_w (f)$ plus a Dirac $delta$ at $f = f_1$. (Specific strength of $delta$ depends on the value of $A$.)
== Variance of the estimator <sec:2-3>
$
variant hat(A) = (H^dagger C^(-1) H)^(-1),
$
which is a scalar. Notice that $H prop A$, so $A^2 variant hat(A)$ does not depend on $A$, which simplifies the problem.
#figure(
image("fig/variance.png", width: 60%),
caption: [
Normalized variance of the BLUE estimator, i.e. $A^2 variant hat(A)$
$N=50$.
]
) <fig:variance>
As shown in @fig:variance, *$f_1 = 1/4$ yields the smallest $variant hat(A)$* for $N=50$.
- The relation between $variant hat(A)$ and $f$ is *similar to PSD* (of $w$) $P_w$ (shown in @fig:PSD).
This should not be too surprising. The less the noise $w$ corrupts the signal, the more precise we are able to estimate $A$.
Extreme case: If $w$ does not intersect with $A cos(2pi f_1 n)$ in frequency domain, then we can estimate $A$ exactly by leveraging a band-pass filter at $f_1$.
- The relation is *symmetric* about $1/4, 0$, and has *period* $1/2$.
The argument for PSD also stands here.
Moreover, we can explain the period $1/2$ in a new way. We estimate $A$ by counting $cos(2pi f_1 m) cos(2pi f_1 n) = 1/2 (cos(2pi f_1 (m+n)) - cos(2pi f_1 (m-n)))$, where $m,n in ZZ$. Note that $m+n, m-n in 2 ZZ$. That is, $hat(A)$ only contains $cos(4pi f_1 ZZ)$. That means if you change $f_1 |-> f_1 + 1/2$, $hat(A)$ does not change, because $4pi times 1/2 = 2pi$ is a period of $cos$.
- $variant hat(A)$ attains *local minimum at $0$ and $1/2$*.
$cos(0 n)$ ($+,+,...$) and $cos(pi n)$ ($+,-,...$) are orthogonal. If $f_1 = 0$, then the band-pass filter of $f_1$ will remove the $f=1/2$ component totally. Similarly, $f_1 = 1/2$ leads to complete removal of $f=0$.
Therefore, $f_1 = 0$ or $1/2$ band-pass filter will remove one of the two components of $w$, so $variant hat(A)$ is about a half of the worst case.
- The curve is *not stable around $0$ and $1/2$*.
The phenomenon exists for any $N$, and the unstable range is more concentrated for larger $N$. (See @fig:variance-ns and @fig:variance-n-500)
#figure(
image("fig/variance-ns.png", width: 70%),
caption: [
$N A^2 variant hat(A)$ for different $N$'s
]
) <fig:variance-ns>
$hat(A)$ is roughly a band-pass filter at $f_1$ applied on $x$ normalized to $A$. If the sequence $cos(2pi f_1 n), space n=0,...,N-1$ does not vary sufficiently, the filter is not well-behaved.
I've discussed the unstable range with 林曦萌, and he explains how the range is unstable. No matter what $C^(-1)$ is, $H^dagger C^(-1) H$ is always a linear combination of $cos(2pi f m) cos(2pi f n)$ (where $m,n in {0,...,N-1}$), and we can regard it as a partial sum of a cosine series. The most unstable component among them is $cos(4pi (N-1) f)$, and it contributes to the unstable range. This explains why the curve oscillates more violently (and therefore more concentrated) for larger $N$.
- $N A^2 variant hat(A) -> 2 P_w (f_1)$ *as $N -> +oo$* for $f_1 in (0,1/2)$ pointwise. (See @fig:variance-n-500)
#figure(
image("fig/variance-n-500.png", width: 80%),
caption: [
$N A^2 variant hat(A)$ for $N = 500$
]
) <fig:variance-n-500>
I have given a qualitative comprehension when I was comparing @fig:variance to @fig:PSD above, and here is a more quantitative (but still not rigorous) comprehension.
PSD $P_w$ is Fourier of ACF $r_(w w)$, which generates the covariance matrix $C$. Let $v$ be the inverse Fourier of $1/P_w$, and it generates another Toeplitz matrix $V$ according to $V_(i j) = v[i-j]$.
_Now I claim that $V -> C^(-1)$ as $N->+oo$._
Reason:
1. By construction of $v$, $r_(w w) * v = delta$, except at where we truncate them.
2. For any vector $y$, $C y = r_(w w) * y$. This is a the motivation behind Toeplitz matrices.
3. Substitute $y[i] = v[i-j]$, we get $C V = I$, except around 4 edges of the matrix.
4. As $N->+oo$, those edges are negligible.
@fig:covariance_and_precision shows $C^(-1)$ for a finite $N$. We can see that $C^(-1)$ is Toeplitz-ish in the center, and fades out around the edges.
#figure(
image("fig/covariance_and_precision.png", width: 80%),
caption: [
$C$ and $C^(-1)$ for $N = 30$
Color of the cell at row $r$ column $c$ represents $C_(r c)$ and $(C^(-1))_(r c)$ respectively.
]
) <fig:covariance_and_precision>
_Second claim: $V H -> 1 / (P_w (f_1)) H$ as $N->+oo$._
Reason:
1. $cos(2pi f_1 n)$ is an eigen-function of any linear shift-invariant system with symmetric frequency response.
2. $v$ represents such a system, and its frequency response is $1/P_w$.
3. $V H = v * H = 1 / (P_w (f_1)) H$ as $N->+oo$.
_Third claim: $2/(N A^2) H^dagger H -> 1$ as $N->+oo$._
Reason: $H^dagger H = sum A^2 cos^2(2pi f_1 n) approx sum A^2/2 = 1/2 N A^2$.
Combining the claims, we have
$
variant hat(A)
&= 1/(H^dagger C^(-1) H)
&-> 1/(H^dagger V H)
&-> 1/(H^dagger 1/(P_w (f_1)) H)
&= (P_w (f_1)) / (H^dagger H)
&-> 2/(N A^2) P_w (f_1),
$
or
$
N A^2 variant hat(A) -> 2 P_w (f_1).
$
= DC level estimation and maximum likelihood estimator
== Theoretical derivations
The average log likelihood
$
(ln p) / N
&= - ln sqrt(2pi sigma^2) - overline((x - A)^2) / (2 sigma^2).
$
The numerator of the second term is the only part depending on $A$, and it can be collected as
$
overline(x^2) - 2 A overline(x) + A^2
&= overline(x^2) - overline(x)^2 + (A - overline(x))^2.
$
Now it is obvious that $hat(A) = overline(x)$ is a maximum likelihood estimator (MLE).
$overline(x)$ follows a normal distribution as it is a linear combination of joint normally distributed $x[0], x[1], ..., x[N-1]$. Then we can find out the distribution by calculating first 2 moments:
$
expect overline(x)
&= overline(expect x) = overline(A) = A. \
variant overline(A)
&= variant (sum x)/N = (variant sum x)/N^2
= (sum variant x)/N^2
= (N sigma^2)/N^2 = sigma^2/N. \
$
Therefore $overline(x) tilde cal(N)(A, sigma^2/N)$.
== Monte--Carlo simulations
Histograms of Monte--Carlo simulations comparing with $cal(N)(A, sigma^2/N)$ are as the following.
#figure(
image("fig/PDF-1000.png", width: 60%),
caption: [
PDF by theory and simulation
$A=1$, $sigma^2=0.1$, $N=50$ with $M=1000$ realizations.
]
) <fig:PDF-1000>
- PDF of $cal(N)(A, sigma^2/N)$ is a *bell curve* centered at $1.00$ with half width around $0.05$ in @fig:PDF-1000.
$A = 1$ and $sqrt(sigma^2/N) approx 0.045$ match the result.
- The simulation shown in @fig:PDF-1000 *verifies* the theoretical distribution.
Histogram in @fig:PDF-1000 does not deviate too much from $cal(N)(A, sigma^2/N)$, because the number of bins is chosen properly by Scott's normal reference rule.
If we split data into too many bins, then data in each bin is not sufficiently large, and unrealistic random fluctuations will appear in the histogram. (See @fig:PDF-1000-too_many_bins)
#figure(
image("fig/PDF-1000-too_many_bins.png", width: 40%),
caption: [
PDF by theory and simulation, but too many bins
The data is identical to that in @fig:PDF-1000.
]
) <fig:PDF-1000-too_many_bins>
This is an essential caveat of Monte--Carlo simulations. To obtain a detailed PDF, we should make more realizations. @fig:PDF-5000 increases $M$ from $1000$ to $5000$, and the data is capable of more bins. The simulated PDF match the theoretical PDF more closely for large $M$ (i.e. more realizations).
#figure(
image("fig/PDF-5000.png", width: 60%),
caption: [
PDF by theory and simulation
$A=1$, $sigma^2=0.1$, $N=50$ with $M=5000$ realizations.
]
) <fig:PDF-5000>
- *$M$ does not affect* the position and width of the bell curve.
$M=1000$ (@fig:PDF-1000) and $M=5000$ (@fig:PDF-5000) are two different simulations for the _same_ distribution. Be aware that we have two dimensionless numbers: $M$ determines accuracy of our _simulation_, and $N$ affects accuracy of the _estimator_.
= DC level estimation and recursive least squares
== Derivations
$x[n] tilde cal(N)(A, r^n)$ independently, so the least squares estimator (LSE)
$
hat(A) = (sum_n r^(-n) x[n]) / (sum_n r^(-n))
$
with variance
$
variant hat(A) = 1 / (sum_n r^(-n)).
$
Let
- $hat(A)$ be LSE for $x[0],...,x[N-1]$, and $hat(A)'$ be LSE for $x[0],...,x[N]$;
- $X := sum_(n<N) r^(-n) x[n]$, and $X' := sum_(n<=N) r^(-n) x[n] = X + r^(-N) x[N]$;
- $S := sum_(n<N) r^(-n)$, and $S' := sum_(n<=N) r^(-n) = S + r^(-N)$.
To find the recursive version
$
hat(A)'
&= X'/S'
= (X + r^(-N) x[N]) / S'
= (S hat(A) + r^(-N) x[N]) / S' \
&= S/S' hat(A) + r^(-N) / S' x[N] \
&= hat(A) + underbrace(r^(-N) / S', "gain factor") (x[N] - hat(A)). \
$
The gain factor
$
K'
&:= r^(-N) / S'
= r^(-N) / (S + r^(-N))
= r^(-N) / (1/(variant hat(A)) + r^(-N)) \
&= (variant hat(A)) / (r^N + variant hat(A)).
$
and
$
variant hat(A)'
&= 1/S'
= r^N K'
= (r^N variant hat(A)) / (r^N + variant hat(A)) \
&= (1 - K') variant hat(A).
$
To wrap up:
- *Estimator update*:
$
hat(A)[n] = underbrace(hat(A)[n-1], "last estimate") + K[n] underbrace((x[n-1] - hat(A)[n-1]), "correction"),
$
where the gain factor
$K[n] = (variant hat(A)[n-1]) / (variant hat(A)[n-1] + r^n)$.
- *Variance update*:
$
variant hat(A)[n] = (1 - K[n]) variant hat(A)[n-1].
$
- *Initial condition*:
- Estimator: $hat(A)[0] = x[0]$.
- Variance: $variant hat(A)[0] = r^0 = 1$.
_Remarks._
In fact it is redundant to iterate all three variable, because $variant hat(A)$ and $K$ are highly related.
To specific, we can merge $variant hat(A)[n-1] |-> K$ and $(variant hat(A)[n-1], K) |-> variant hat(A)[n]$.
Moreover, it would be even simpler to iterate $X,S$ in previous derivation.
== Simulations
$variant hat(A)$ and $K$ does not change across simulations after $A, r$ are determined. We will analyze $hat(A)$ after talking about $variant hat(A)$ and $K$.
#figure(
image("fig/recursive-variance.png", width: 100%),
caption: [
Variance $variant hat(A)$ by $n$ iterations
The right plot is a zoom of the left.
]
)
- $variant hat(A)$ *decreases* as $n$ increases.
The larger $N$ is, the more we know about $A$, and the better we can estimate it.
In addition, $variant hat(A)$ *drops faster* when $n$ is small. New estimation is a compromise between last estimation and new data. When $n$ is small, existing estimation is weakly trusted, so new data matters more, and reduces $variant hat(A)$ more significantly.
- $variant hat(A)$ is larger for *larger $r$*. (when $n$ is the same)
Larger $r$ means $w$ with larger variance. It infers that $x$ is more contaminated, making $A$ harder be to estimate.
- $variant hat(A)$ would be *saturated* after some iterations if $r > 1$.
As $n -> +oo$,
- $abs(r) > 1$: $variant hat(A) = 1 \/ sum r^(-n) -> r-1 > 0$.
- $r = 1$: $variant hat(A) = 1/(n+1) -> 0$.
- $r in (0,1)$: $variant hat(A) = 1 \/ sum_(n'<n) r^(-n') = (1-r) / (1-r^(-n)) = order(r^n) -> 0$.
- $variant hat(A)$ is always *positive*.
It is nonnegative by definition. It is nonzero because the initial randomness can never be erased.
#figure(
image("fig/recursive-gain.png", width: 100%),
caption: [
Gain factor $K$ by $n$ iterations
The right plot is a zoom of the left.
]
)
- $K$ *decreases* as $n$ increases.
Again, new estimation is a compromise between last estimation and new data. Once $n$ is sufficiently large, we have obtained almost all the information of $A$ from existing data, so new data matters less.
In addition, $K$ *drops faster* when $n$ is small.
- $K$ is larger for *smaller $r$*. (when $n$ is the same)
When $r$ is smaller, the variance of $w$ decreases faster (or increases slower). Therefore, new data is more accurate and matters more.
- $K$ does *not converge to zero* if $r < 1$.
If $r in (0,1)$, then the variance of $w$ decreases, so new data is always better than all existing data, so it is wise to take new data into consideration.
Quantitatively, as $n -> +oo$,
- $abs(r) > 1$: $K = r^(-n) variant hat(A) = r^(-n) times order(1) -> 0$.
- $r = 1$: $K = 1 times variant hat(A) -> 0$.
- $r in (0,1)$: $K = r^(-n) / (sum r^(-n)) = 1 \/ sum r^n -> 1-r > 0$.
- $K$ is always strictly *between $0$ and $1$*.
New estimation is a compromise between last estimation and new data with weight $(1-K):K$. To make sure that new estimation is between last estimation and new data, both $1-K$ and $K$ should be nonnegative, and thus $K in [0,1]$.
If $K$ takes one of the endpoints, then either existing data or new data is totally ignored. Insufficient use of data never gives a good estimator.
Now let us discuss $hat(A)$, which is a random variable (or a random sequence). The simulation result is shown in @fig:recursive-estimator.
#figure(
image("fig/recursive-estimator.png", width: 80%),
caption: [
Estimator $hat(A)$ by $n$ iterations for different $r$'s
$A=10$.
]
) <fig:recursive-estimator>
- $x$ is roughly distributed *between* $A plus.minus r^(n\/2)$.
It is a feature of $cal(N)(A, r^n)$.
- $r in (0,1)$: Newer data has less noise.
- $r = 1$: The contamination does not depend on $n$.
- $r > 1$: Only first several data concentrated around the true value $A=10$, and later data tends to diverge to the whole $RR$.
- $hat(A)$ never goes wildly no matter how large $r$ is, and *converges* if $r <= 1$.
It is due to the trend of $variant hat(A)$.
Even if $r > 1$, $hat(A)$ does not variate as wildly as $x$ does, because polluted data is ignored.
- $hat(A)$ approaches to the true value more *quickly* for smaller $r$.
It is a subtle phenomenon, and worth pointing out evidences. For $r=0.95$, the line of $hat(A)$ coincides with the horizontal grid line of $10$ starting from $n approx 60$. For $r=1$, it coincides starting from $n approx 90$. For $r=1.05$, it never coincides.
It also due to the trend of $variant hat(A)$.
- For $r>1$, $hat(A)$ can get stuck at a *biased* value.
In this case, later data is too contaminated to be used effectively, so the major factor of $hat(A)$ is only the first several data. Needless to say, such a small sample size can hardly yields a good estimation.
- Different $r$'s are indistinguishable in the *beginning*.
(This is an alternative question of estimating $r$ with $A$ known or unknown.)
When $n$ is small (say, $n < 10$), all 3 cases gives unstable estimations with large error.
$r$ does not alter $r^n$ too much if $n$ is small, so they are indistinguishable. There is too few data if $n$ is small, hence insufficient information, so all estimations are bad.
= Comparison between methods
1. *CRLB* is a good lower bound, but sometimes it cannot give a specific estimator.
2. *BLUE* is viable (at least numerically) if the first two moments of samples is known. We can asses its performance in terms of first two moments, but we are not certain about whether better nonlinear estimator exists.
3. *MLE* can be performed if we assume the PDF of samples. We cannot tell if it is biased or calculate the variance for a finite sample size, but we can claim asymptotical properties: MLE is asymptotically unbiased, efficient, and consistent. Monte--Carlo method tells how the limit fast is for specific true values.
4. *LSE* is a practical estimator with no theoretical assurance, featuring the possibility of monitoring --- We can estimate sequentially and decide what to do next, while other estimators are waiting for the full data.
In all four experiments, the quality of data is crucial for estimation. We can never estimate robustly if *noise* overwhelms the *signal* representing parameters.
The relation is not restricted not single numbers (variances for example). As discussed in @sec:2-3, feature spaces such as frequency domain may also matter.
|
|
https://github.com/hekzam/typst-lib | https://raw.githubusercontent.com/hekzam/typst-lib/main/test/basic.typ | typst | Apache License 2.0 | #import "../lib.typ": rect-box, gen-copies
#let bcol = json("./input-fill-colors.json")
#let f-color = white
#let copy-two-pages = int(sys.inputs.at("copy-two-pages", default: "0")) == 1
#let copy-content = [
#set align(left+top)
= Exercise 1
#rect-box("b0", 5cm, 1cm, fill-color: color.rgb(bcol.at("b0")))
Inline #box(rect-box("b0i", 8cm, 2.5cm, fill-color: color.rgb(bcol.at("b0i"))))
#rect-box("b1", 100%, 1cm, fill-color: color.rgb(bcol.at("b1")))
#if copy-two-pages {
pagebreak()
}
= Exercise 2
#rect-box("b2", 5cm, 1cm, fill-color: color.rgb(bcol.at("b2")))
Inline #box(rect-box("b2i", 8cm, 2.5cm, fill-color: color.rgb(bcol.at("b2i"))))
#set align(bottom)
= Exercise 3
This exercise should be at the bottom of its page.
#rect-box("b3", 100%, 1cm, fill-color: color.rgb(bcol.at("b3")))
]
#let n = int(sys.inputs.at("nb-copies", default: "1"))
#let d = int(sys.inputs.at("duplex-printing", default: "0")) == 1
#gen-copies("basic", copy-content, nb-copies: n, duplex-printing: d)
|
https://github.com/kotfind/hse-se-2-notes | https://raw.githubusercontent.com/kotfind/hse-se-2-notes/master/edu/homeworks/book_table/main.typ | typst | #set text(lang: "ru", size: 11pt)
#set par(justify: true)
#align(center)[
#text(size: 20pt, weight: "bold")[Ответы на вопросы\ по книге "<NAME>"\ Кендзиро Хайтани]
#text(size: 18pt)[<NAME>\ БПИ233]
]
#text(weight: "bold", size: 14pt)[
Как вы думаете, почему японская пословица "Не нужно быть Буддой, чтобы
взглянуть в глаза кролику и увидеть мир его глазами" дала название повести
“Взгляд кролика?” Подкрепите свои мысли идеями из книги.
]
Пословица говорит о возможности одного человека понять мировоззрение и ценности
другого. О том же говорится в книге Кендзиро Хайтани: многие учителя не
понимают и не пытаются понять своих учеников. Они обвиняют детей в
нечистоплотности, говорят, что те "не люди вовсе"#footnote[Гл. 3],
не боясь задеть их чувства.
Тем не менее, Адачи-сенсей, а впоследствии и остальная _четверка_ (так я буду
называть Адачи, Орихаши, Ота и Котани), показывают обратный пример: они много
общаются с ребятами, пытаются их понять и прикладывают все возможные усилия,
чтобы помочь в беде.
#text(weight: "bold", size: 14pt)[
Что отличает Фуми Котани от других учителей в школе? Приведите 2-3 примера
]
Если сравнивать Котани с основной частью преподавательского состава, то отличия
сразу же бросаются в глаза.
+ *Котани пытается понять своих учеников.* Она ходит к школьникам домой и на
"базу", общается с ребятами и поддерживает их в трудную минуту (например,
помогает собрать денег, работая старьевщиком#footnote[Гл. 19]).
Фуми вводит систему дневников, которая позволяет ей больше узнавать о своих
учениках #footnote[Гл. 16]. Пытаясь найти общий язык с Тэцуо сенсей сама
начинает изучать книги про мух#footnote[Гл. 6].
+ *Котани верит в своих учеников, уважает их мнение и прислушивается к нему.*
Когда из-за прихода Минако, в классе возникают проблемы, Фуми, не зная что
делать, созывает собрание и просит у своих учеников совета.
Она выслушивает идею Джуна и делает так, как он и предложил#footnote[Гл. 12].
+ *Котани любит своих учеников и свою работу.* Несмотря, на различные трудности
и усталость, она продолжает преподавать и стремится быть хорошим учителем.
Она не соглашается, когда её отец говорит, что зря предложил дочери
устроится в школу#footnote[Гл. 24], и очень расстраивается, когда её муж
просит поскорее прогнать детей, пришедших навестить учительницу#footnote[Гл. 17].
// TODO: сравнить с четверкой
#text(weight: "bold", size: 14pt)[
Опишите "идеального ученика" и "ужасного ученика" по мнению школьного
коллектива. Приведите 2-3 примера.
]
"Ужасными" по мнению школьного коллектива являются нечистоплотные дети (про это
говорит Мурано-сенсей, вспоминая Кодзи, и другие учителя, вспоминая разводящего
мух Тэцуо#footnote[Гл. 4]), дети работников мусорного завода.
"Хорошими" же считаются дети подавленные --- как минимум, такое обвинение
Адачи-сенсей направляет в адрес других учителей: "Задумайтесь, коллеги, и честно ответьте себе на один простой
вопрос: а не выходит ли так, что забота о здоровье ребенка — это всего лишь предлог для
того, чтобы этого самого ребенка подавить, унизить, продемонстрировать ему свое
презрение?"#footnote[Гл. 4].
#text(weight: "bold", size: 14pt)[
Напишите, с какими основными трудностями сталкивается Фуми Котани во время
работы в школе? Видят ли эти же трудности другие учителя? В чем разница в их
подходе решения этих проблем? Приведите 2-3 примера
]
+ *Котани сталкивается с проблемой сложных учеников.* К таковым можно отнести
Тэцуо и Минако.
+ В случае Тэцуо, Фуми пытается лучше понять мальчика. Узнать больше о нем,
о его увлечениях. Другие же учителя просто считали его странным,
агрессивным и ничего не предпринимали.
+ В случае с Минако, сенсей пыталась социализировать девочку. Она объясняла
другим детям, что Минако хорошая и что не стоит на неё
обижаться#footnote[Гл. 11]. Другие учителя и некоторые родители хотели
изолировать девочку от других детей и перевести её в специальное учебное
заведение.
+ Притом, что другие дети (например, Кодзи) были совершенно обычными, у некоторых
учителей были с ними проблемы. Учителя обзывали и не поддерживали их,
в целом плохо к ним относились. У Котани таких проблем не было, она
просто относилась к ребятам по-человечески.
#text(weight: "bold", size: 14pt)[
Через какие техники/приемы у Фуми Котани получается найти подход к "трудным
детям"? Приведите 2-3 примера.
]
+ *Котани пытается понять своих учеников.* _Смотри одноименный пункт в вопросе 2._
+ *Котани обращается за советом к Адачи, учится на его примере.*
Особенно в начале книги, Фуми часто обращается за помощью к Адачи -- более
опытному учителю чем она. Она также замечает, что дети любят
сенсея#footnote["И почему они его так любят?" --- с досадой подумала Котани-сэнсей., Гл. 2] и пытается найти
причину этому.
+ *Котани обращается за советом к самим ребятам.* Ребята хорошо знают друг
друга, а также, как и взрослые, имеют своё мнение. Так, попадая в сложную
ситуацию из-за Минако, сенсей обсуждает проблему с классом. _Подробнее смотри
пункт 2 в вопросе 2._
#line(length: 100%)
*P.S.* Спасибо большое за книжку, очень хорошая!!!
Мне очень понравились четверо "основных" учителей, особенно, Адачи-сенсей. На
протяжении всей книги было ощущение, что Адачи напоминает мне персонажа из
какого-то другого произведения: до сих пор не могу точно понять, кого именно.
Возможно, Онидзуку, впрочем я не уверен.
|
|
https://github.com/wenjia03/JSU-Typst-Template | https://raw.githubusercontent.com/wenjia03/JSU-Typst-Template/main/Templates/实验报告(无框)/template/body.typ | typst | MIT License | #import "../utils/style.typ": *
#import "../utils/utils.typ": *
#import "../config/thesisInfo.typ": *
#import "@preview/cuti:0.2.0": show-cn-fakebold
#show: show-cn-fakebold
#pagebreak()
#set page(numbering: "1")
#counter(page).update(1)
// 章节计数器,记录公式层级
// -----------------
// 2023/4/11 update log:
// - 增加计数器,把元素序号转为 `x.x` 的格式
#let counter_chapter = counter("chapter")
#let counter_equation = counter(math.equation)
#let counter_image = counter(figure.where(kind: image))
#let counter_table = counter(figure.where(kind: table))
// 图片和表的格式
// -----------------
// 2023/4/11 update log:
// - 把元素序号转为 `x.x` 的格式
#show figure: it => [
#set text(font_size.wuhao)
#set align(center)
#if not it.has("kind") {
it
} else if it.kind == image {
it.body
[
#textbf("图")
#locate(loc => {
[#counter_chapter.at(loc).first().#counter_image.at(loc).first()]
})
#it.caption
]
} else if it.kind == table {
[
#textbf("表")
#locate(loc => {
[#counter_chapter.at(loc).first().#counter_table.at(loc).first()]
})
#it.caption
]
it.body
} else {
it.body
}
]
// 设置公式格式
// -----------------
// 2023/4/11 update log:
// - 修复为章节号 + 公示编号
#set math.equation(
numbering: (..nums) => locate( loc => {
numbering("(1.1)", counter_chapter.at(loc).first(), ..nums)
})
)
// 设置引用格式
// -----------------
// 2023/4/11 update log:
// - 原本对公式的引用是 `Equation (x.x)`,改为 `式x.x`
#show ref: it => {
locate(loc => {
let elems = query(it.target, loc)
if elems == () {
it
} else {
let elem = elems.first()
let elem_loc = elem.location()
if numbering != none {
if elem.func() == math.equation {
link(elem_loc, [#textbf("式")
#counter_chapter.at(elem_loc).first().#counter_equation.at(elem_loc).first()
])
} else if elem.func() == figure{
if elem.kind == image {
link(elem_loc, [#textbf("图")
#counter_chapter.at(elem_loc).first().#counter_image.at(elem_loc).first()
])
} else if elem.kind == table {
link(elem_loc, [#textbf("表")
#counter_chapter.at(elem_loc).first().#counter_table.at(elem_loc).first()
])
}
}
} else {
it
}
}
})
}
#set heading(numbering: (..nums) =>
if nums.pos().len() == 1 {
zhnumbers(nums.pos().first()) +"、"
}
else {
nums.pos().map(str).join(".")
})
#show heading: it => {
if it.level == 1 {
// set align(center)
set text(font:字体.黑体, size: 字号.小四, weight: "bold")
counter_chapter.step()
counter_equation.update(())
counter_image.update(())
counter_table.update(())
it
} else if it.level == 2 {
set text(font:字体.黑体, size: 字号.小四, weight: "bold")
it
par(leading: 1.5em)[#text(size:0.0em)[#h(0.0em)]]
} else if it.level == 3 {
set text(font:heiti, size: font_size.xiaosi, weight: "regular")
it
par(leading: 1.5em)[#text(size:0.0em)[#h(0.0em)]]
}
}
// 设置正文格式
#set text(font: 字体.宋体, size: 字号.小四)
#set par(justify: false, leading: 1.5em, first-line-indent: 2em)
#show par: it => {
it
v(5pt)
}
#let title(titles) = {
set align(center)
text(font:字体.黑体, size: 字号.小二, weight: "regular",titles)
}
#title("实验"+zhnumbers(experimentId)+" "+experimentName)
#include "../contents/context.typ"
|
https://github.com/francescoo22/kt-uniqueness-system | https://raw.githubusercontent.com/francescoo22/kt-uniqueness-system/main/src/annotation-system/rules/unification.typ | typst | #import "../../proof-tree.typ": *
#import "../../vars.typ": *
// *********** Unify ***********
#let Ctx-Lub-Empty = prooftree(
axiom($$),
rule(label: "Ctx-Lub-Empty", $dot space lub space dot space = space dot$),
)
#let Ctx-Lub-Sym = prooftree(
axiom($$),
rule(label: "Ctx-Lub-Sym", $Delta_1 lub Delta_2 = Delta_2 lub Delta_1$),
)
#let Ctx-Lub-1 = prooftree(
axiom($Delta_2 inangle(p) = alpha'' beta''$),
axiom($Delta_2 without p = Delta'_2$),
axiom($Delta_1 lub Delta'_2 = Delta'$),
axiom($Lub{alpha beta, alpha'' beta''} = alpha' beta'$),
rule(n:4, label: "Ctx-Lub-1", $(p : alpha beta, Delta_1) lub Delta_2 = p : alpha' beta', Delta'$),
)
#let Ctx-Lub-2 = prooftree(
axiom($x in.not Delta_2$),
axiom($Delta_1 lub Delta_2 = Delta'$),
rule(n:2, label: "Ctx-Lub-2", $(x : alpha beta, Delta_1) lub Delta_2 = x : top, Delta'$),
)
#let Remove-Locals-Base = prooftree(
axiom($$),
rule(label: "Remove-Locals-Base", $dot triangle.filled.small.l Delta = dot$),
)
#let Remove-Locals-Keep = prooftree(
axiom($root(p) = x$),
axiom($Delta_1 inangle(x) = alpha' beta'$),
axiom($Delta triangle.filled.small.l Delta_1 = Delta'$),
rule(n:3, label: "Remove-Locals-Keep", $p : alpha beta, Delta triangle.filled.small.l Delta_1 = p : alpha beta, Delta'$),
)
#let Remove-Locals-Discard = prooftree(
axiom($root(p) = x$),
axiom($x in.not Delta_1$),
axiom($Delta triangle.filled.small.l Delta_1 = Delta'$),
rule(n:3, label: "Remove-Locals-Discard", $p : alpha beta, Delta triangle.filled.small.l Delta_1 = Delta'$),
)
#let Unify = prooftree(
axiom($Delta_1 lub Delta_2 = Delta_lub$),
axiom($Delta_lub triangle.filled.small.l Delta = Delta'$),
rule(n:2, label: "Unify", $unify(Delta, Delta_1, Delta_2) = Delta'$),
)
// *********** Normalize ***********
#let N-Empty = prooftree(
axiom($$),
rule(label: "N-Empty", $norm(dot) = dot$)
)
#let N-Rec = prooftree(
axiom($Lub(alpha_i beta_i | p_i = p_0 and 0 <= i <= n) = ablub$),
axiom($norm(p_i: alpha_i beta_i | p_i != p_0 and 0 <= i <= n) = p'_0 : alpha'_0 beta'_0, ..., p'_m : alpha'_m beta'_m$),
rule(n:2, label: "N-rec", $norm(p_0\: alpha_0 beta_0, ..., p_n\: alpha_n beta_n) = p_0 : ablub, p'_0 : alpha'_0 beta'_0, ..., p'_m : alpha'_m beta'_m$)
)
|
|
https://github.com/astrale-sharp/typst-assignement-template | https://raw.githubusercontent.com/astrale-sharp/typst-assignement-template/main/libs/expression.typ | typst | MIT License | #import "science.typ" : sfmt
#let node(label, value) = {
assert(type(label)=="string")
assert(type(value) in ("float","integer"))
(label : label, value : value, type : "node")
}
// #let is_node(node) = {
// type(node)=="dictionary" and node.type == "node"
// }
#let show_node(node) = {
eval("$" + node.label + "=" + str(node.value) + "$")
}
#let expr_to_val(..nodes) = {
// args must be string or node
assert(nodes.pos().all(i => type(i) == "string" or is_node(i)))
let v = "#{" + nodes.pos().map(i => if is_node(i) {str(i.value)} else {i}).join("") + "}"
v
}
#let expr_to_label(..nodes) = {
// args must be string or node
assert(nodes.pos().all(i => type(i) == "string" or is_node(i)))
let s = nodes.pos().map(i => if is_node(i) {i.label} else {i}).join(" ")
s.replace("*","times")
}
#let expr_to_dev(..nodes) = {
// args must be string or node
assert(nodes.pos().all(i => type(i) == "string" or is_node(i)))
let s = nodes.pos().map(i => if is_node(i) {str(i.value)} else {i}).join(" ")
s.replace("*","times")
}
#let a = node("P_a",700.2);//#show_node(a)
#let b = node("P_d",5);//#show_node(b)
#let expression(
x,
nodes : (),
digit : none,
show_labels : true,
show_values : true,
show_result : true
) = {
let matches = x.matches(regex(":\\w"))
let mathematize(x) = "$" + x +"$"
let replace(value,q) = {
let res = value
for k in matches {
let tok = k.text
res = res.replace(tok,str(q(nodes.at(tok.trim(":")))))
}
res
}
let labels = if show_labels {
replace(x,i=> i.label).replace("*","times") + " = " } else {""}
let values = if show_values {
replace(x,i=> i.value).replace("*","times")
} else {""}
let result = if show_result {
let tmp = replace(x,i=> i.value)
for m in tmp.matches(regex("(\\w|\\a)+\\^(\\w|\\a)+")) {
tmp = tmp.replace(m.text, "calc.pow(" + m.text.split("^").at(0) +"," + m.text.split("^").at(1) + ")")
}
let tmp = eval(tmp)
" = " + sfmt(
number_of_digit : digit,
tmp
)
} else {""}
eval(mathematize(labels + values)) + result
}
On considère que
#let a = node("P_\"some\"",10);#show_node(a) m.s
et
#let b = node("d",5158);#show_node(b) m
#let e = expression.with(
nodes : ("a" : a,"b" : b)
)
#e(
digit : 0,
":a^2 * :a^2",
)
#e(":a") m
// #r(
// "(:a + :b)/ (:b) - 5"
// )
// #e3(
// "(:a * :b)/ (:b) - 5",
// )
|
https://github.com/rem3-1415926/Typst_Thesis_Template | https://raw.githubusercontent.com/rem3-1415926/Typst_Thesis_Template/main/sec/appendix.typ | typst | MIT License |
#let appendix = [
#include("../appendix/app1.typ")
#include("../appendix/app2.typ")
#include("../appendix/app3.typ")
] |
https://github.com/jgm/typst-hs | https://raw.githubusercontent.com/jgm/typst-hs/main/test/typ/compute/calc-05.typ | typst | Other | // Error: 8-15 invalid float: 1.2.3
#float("1.2.3")
|
https://github.com/swouf/alina-poster-template | https://raw.githubusercontent.com/swouf/alina-poster-template/main/README.md | markdown | Creative Commons Attribution Share Alike 4.0 International | # Alina Poster Template
This is a simple and naive template for making academic posters with Typst.
This is an ongoing, unfinished project. Use it at your own risk.
Feel free to modify it and adapt it. Please, mind the _CC-BY-SA 4.0_ license and respect the terms of it (respect attribution and share any derivatives under a compatible license).
## How to use this template?
Clone the repo or just copy the main file, `alina-poster.typ`. Then, import it in your poster.
```typst
#import "alina-poster.typ": alina_poster, alina_block, alina_ammo_bar, alina_chip, alina_highlight, palette
```
Finally, to create the poster, you can initiate it as any normal Typst template.
```typst
#show: doc => alina_poster(
title: [Your title.],
authors: (
(
name: "<NAME>",
email: "<EMAIL>",
affiliation: "École Polytechnique Fédérale de Lausanne",
correspondingAuthor: true,
),
),
topAbstract: [
Your abstract.
],
footer: footer,
body: block(inset: 32pt, stack(dir: ttb, doc)),
)
```
### Why "Alina"?
It's simply the first name that came to my mind when choosing a name for this project. Next template will start with a B.
<p xmlns:cc="http://creativecommons.org/ns#" xmlns:dct="http://purl.org/dc/terms/"><a property="dct:title" rel="cc:attributionURL" href="https://github.com/swouf/alina-poster-template">Alina Poster Template</a> by <a rel="cc:attributionURL dct:creator" property="cc:attributionName" href="https://github.com/swouf"><NAME></a> is licensed under <a href="https://creativecommons.org/licenses/by-sa/4.0/?ref=chooser-v1" target="_blank" rel="license noopener noreferrer" style="display:inline-block;">Creative Commons Attribution-ShareAlike 4.0 International<img style="height:22px!important;margin-left:3px;vertical-align:text-bottom;" src="https://mirrors.creativecommons.org/presskit/icons/cc.svg?ref=chooser-v1" alt=""><img style="height:22px!important;margin-left:3px;vertical-align:text-bottom;" src="https://mirrors.creativecommons.org/presskit/icons/by.svg?ref=chooser-v1" alt=""><img style="height:22px!important;margin-left:3px;vertical-align:text-bottom;" src="https://mirrors.creativecommons.org/presskit/icons/sa.svg?ref=chooser-v1" alt=""></a></p> |
https://github.com/FlandiaYingman/typstfmt-ext | https://raw.githubusercontent.com/FlandiaYingman/typstfmt-ext/master/README.md | markdown | # typstfmt Ext
A Chromium extension that calls `typstfmt` on the current [Typst App](https://typst.app/) Document!
> [!CAUTION]
> This extension is in early development and may break your document. Backup your work before using it.
## Installation
> [!NOTE]
> I have submitted the extension
> to [Edge Add-ons](https://microsoftedge.microsoft.com/addons/Microsoft-Edge-Extensions-Home), but it is still under
> review. For now, you can install it manually.
1. Clone this repository.
2. Turn on "Developer Mode" in `edge://extensions/`.
3. Click "Load Unpacked" and select the `typstfmt-ext` directory.
4. The extension should now be installed.
To use the extension, the `typstfmt` executable must be installed on your system. You can install
it [here](https://github.com/astrale-sharp/typstfmt).
After installing the extension, you have to install
a [Native Messaging Host](https://learn.microsoft.com/en-us/microsoft-edge/extensions-chromium/developer-guide/native-messaging)
to communicate with the `typstfmt` executable. For Windows users, I've already compiled the host and included it in
the `host` directory. For other platforms, you can compile the host yourself with `cmake` and the
included `CMakeLists.txt`
Next, register the host. You can either follow the
instructions [here](https://learn.microsoft.com/en-us/microsoft-edge/extensions-chromium/developer-guide/native-messaging#step-3-register-the-native-messaging-host) (
refer to the following JSON)
or run the `host/register.ps1` file to do so.
```json
{
"path": "<PATH_TO_HOST_EXECUTABLE>",
"description": "TypeScript Formatter Extension Host",
"name": "dev.flandia.typstfmt_ext_host",
"allowed_origins": [
"chrome-extension://ebkbgnmkkebikgiohnipickpecmcilep/"
],
"type": "stdio"
}
```
Finally, if you registered the host by running `host/register.ps1`, open the generated `manifest.json` file and replace
the `"chrome-extension://ebkbgnmkkebikgiohnipickpecmcilep/"` with the ID of the extension on your machine.
> [!NOTE]
> Since the extension is not yet published, the ID of the extension is not determined. The `allowed_origins` field is
> set to the ID of the extension on my machine. The ID of the extension on your machine will be different. You can find
> it in `edge://extensions/` after installing it.
## Usage
Open a document in [Typst App](https://typst.app/), click the extension button and your document should be formatted!
|
|
https://github.com/ryuryu-ymj/mannot | https://raw.githubusercontent.com/ryuryu-ymj/mannot/main/docs/doc-template.typ | typst | MIT License | #import "/src/lib.typ": *
#let entrypoint = toml("/typst.toml").package.entrypoint
#let usage = "#import \"/" + entrypoint + "\": *\n" + "#show: mannot-init\n"
#let example(source) = {
grid(
columns: (2fr, 1fr),
rows: (auto),
align: center + horizon,
gutter: 5pt,
{
set text(0.8em)
raw(block: true, lang: "typst", source)
},
rect(
width: 100%,
inset: 10pt,
eval(usage + source, mode: "markup"),
),
)
}
|
https://github.com/NOOBDY/formal-language | https://raw.githubusercontent.com/NOOBDY/formal-language/main/template.typ | typst | The Unlicense | #let project(title: "", authors: (), date: none, body) = {
// Set the document's basic properties.
set document(author: authors, title: title)
set page(numbering: "1", number-align: center)
set text(font: "<NAME>", lang: "en")
// Title row.
align(center)[
#block(text(weight: 700, 1.75em, title))
#v(1em, weak: true)
#date
]
// Author information.
pad(
top: 0.5em,
bottom: 0.5em,
x: 2em,
grid(
columns: (1fr,) * calc.min(3, authors.len()),
gutter: 1em,
..authors.map(author => align(center, strong(author))),
),
)
// Main body.
set par(justify: true)
let enum_levels = ("1.", "(a)")
let enum_depth = state("enum_depth", 0)
show enum: it => {
locate(loc => {
let depth = enum_depth.at(loc)
set enum(numbering: enum_levels.at(depth))
enum_depth.update(x => x + 1)
it
enum_depth.update(x => x - 1)
})
}
body
}
|
https://github.com/linhduongtuan/BKHN-Thesis_template_typst | https://raw.githubusercontent.com/linhduongtuan/BKHN-Thesis_template_typst/main/contents/info.typ | typst | Apache License 2.0 | // Title in Vietnamese
#let vn_title = "CÁCH SỬ DỤNG CHATGPT ĐỂ HỖ TRỢ VIẾT LUẬN VĂN BẰNG TIẾNG ANH?"
// Title in English
#let en_title = "HOW TO USE CHATGPT TO IMPROVE THE QUALITY OF A THESIS WRITTEN IN ENGLISH?"
// College/School/Faculty
#let college = "SoICT"
// Major/Discipline
#let major = "Computer Science"
// Student ID
#let student_id = "1912190000"
// Student Name
#let student_name = "<NAME>"
// Advisor No. 1
#let college_advisor = "ABC"
// advisor No. 2
#let company_advisor = "XYZ"
// Start time
#let start_and_end_date = "From 10/06/2019 to 06/06/2023" |
https://github.com/typst/packages | https://raw.githubusercontent.com/typst/packages/main/packages/preview/quill/0.2.0/README.md | markdown | Apache License 2.0 | <h1 align="center">
<img alt="Quantum Circuit" src="docs/images/logo.svg" style="max-width: 100%; width: 300pt">
</h1>
**Quill** is a package for creating quantum circuit diagrams in [Typst](https://typst.app/).
Repository: https://github.com/Mc-Zen/quill
_Note, that this package is in beta and may still be undergoing breaking changes. As new features like types and scoped functions will be added to Typst, this package will be adapted to profit from the new paradigms._
_Meanwhile, we suggest importing everything from the package in a local scope to avoid polluting the global namespace (see example below)._
## Usage
Create circuit diagrams by calling the function `quantum-circuit()` with any number of positional arguments — just like the built-in Typst functions `table()` or `grid()`. A variety of different gate and instruction commands are available and plain numbers can be used to produce any number of empty cells just filled with the current wire style. A new wire is started by adding a `[\ ]` item.
```typ
#{
import "@preview/quill:0.2.0": *
quantum-circuit(
lstick($|0〉$), gate($H$), ctrl(1), rstick($(|00〉+|11〉)/√2$, n: 2), [\ ],
lstick($|0〉$), 1, targ(), 1
)
}
```
<h3 align="center">
<img alt="Bell Circuit" src="docs/images/bell.svg">
</h3>
As a shorthand for simple gates you can also just write `$H$` instead of the more lengthy `gate($H$)`.
Refer to the [user guide](docs/guide/quill-guide.pdf) for a full documentation of this package.
## Gallery
<h3 align="center">
<img alt="Gallery" src="docs/images/gallery.svg" />
</h3>
## Examples
Some show-off examples, loosely replicating figures from [Quantum Computation and Quantum Information by M. Nielsen and <NAME>](https://www.cambridge.org/highereducation/books/quantum-computation-and-quantum-information/01E10196D0A682A6AEFFEA52D53BE9AE#overview).
<h3 align="center">
<img alt="Quantum teleportation circuit" src="docs/images/teleportation.svg">
</h3>
<h3 align="center">
<img alt="Quantum circuit for phase estimation" src="docs/images/phase-estimation.svg">
</h3>
<h3 align="center">
<img alt="Quantum fourier transformation circuit" src="docs/images/qft.svg">
</h3>
## Changelog
### v0.2.0
- New features:
- Arbitrary labels to any `gate` (also derived gates such as `meter`, `ctrl`, ...), `gategroup` or `slice` that can be anchored to any of the nine 2d alignments.
- Add optional gate inputs and outputs for multi-qubit gates (see gallery).
- Implicit gates (breaking change ⚠️): a content item automatically becomes a gate, so you can just type `$H$` instead of `gate($H$)` (of course, the `gate()` function is still important in order to use the many available options).
- Other breaking changes ⚠️:
- `slice()` has no `dx` and `dy` parameters anymore. Instead, labels are handled through `label` exactly as in `gate()`. Also the `wires` parameter is replaced with `n` for consistency with other multi-qubit gates.
- Swap order of row and column parameters in `annotate()` to make it consistent with built-in Typst functions.
- Improvements:
- Improve layout (allow row/column spacing and min lengths to be specified in em-lenghts).
- Automatic bounds computation, even for labels.
- Improve meter (allow multi-qubit gate meters and respect global (per-circuit) gate padding).d
- Fixes:
- `lstick`/`rstick` braces broke with Typst 0.7.0.
- `lstick`/`rstick` bounds.
- Documentation
- Add section on creating custom gates.
- Add section on using labels.
- Explain usage of `slice()` and `gategroup()`.
<!-- - Add Tips and tricks section -->
### v0.1.0
Initial Release |
https://github.com/tairahikaru/old-typst-japanese | https://raw.githubusercontent.com/tairahikaru/old-typst-japanese/main/README.typ | typst | Other | // README.typ
// https://github.com/tairahikaru/old-typst-japanese
// This file is CC BY-SA 4.0
//
// Require Typst 0.2.0 (23/04/11)
#let 現在 = "2023年4月19日現在"
#let LaTeX = { // based on LaTeX kernel
let body = {
[L]
h(-0.36em)
box(height:1em,align(top, smallcaps([a])))
h(-0.15em)
[T]
h(-0.1667em)
text(baseline: 0.2152775em)[E]
h(-0.125em)
[X]
}
style(styles => {
let size = measure(body, styles)
box(width: size.width, place(dy: -size.height, body))
})
}
#show link: it => {
set text(fill:
if type(it.dest) == "string" {
blue
} else {
cmyk(0%,100%,0%,0%)
}
)
it
}
#set raw(lang: "typ")
#import "otypjc.typ": conf, tate
#show: conf.with(
title: "old-typst-japanese README",
author: "tairahikaru",
date: "2023-04-19",
abstract: [
#link("https://github.com/typst/typst")[Typst]#" "で比較的まともに日本語を扱うための#" "
#{現在}#strong[最新]のモジュール。
Typst 0.2.0#" " (23/04/11) 以降が要求される。
],
paper: "jis-b5",
columns-number: 2,
size: 10pt,
)
#outline()
= otypjp
日本語を扱うための基本的な設定をするモジュール。
#{現在}のTypstは日本語組版のためには到底機能不足のため、その場限りの対処を多く行ってる。
ユーザがパラメータを上書きした場合、予期せぬ挙動を引き起こす可能性が大いにある。
== 約物
句読点がつねにぶら下がりになる。括弧類は大丈夫だった。
連続した「約物」(句読点や括弧などのこと)の間の空白の大きさが、
一般的な日本語組版の用件に沿うようになっている。
これは、#text(spacing:100%)[chws (Contextual Half-width Spacing) ]というOpenType featureに
よって実現されており、この機能に対応していない和文フォントを用いた場合は
調整されない。
これに対応した和文フォントとしては、例えば
#link("https://github.com/simonsmh/notocjk/tree/master/system/fonts")[ここから手に入るNotoフォント]
などがある。
Typstは#" "#{現在}#link("https://github.com/typst/typst/issues/185")[#" "variableフォントに
対応していない]ので、先のリンクから入手できるフォントだけでは日本語フォントのRegular以外のウェイトを
利用することはできない。
「括弧類」は、行頭全角二分、折り返し二分。
(約物だけの幅が半角であるようなchws対応のフォントを作れば良いのだろうが、そんなOpenTypeフォントは
見たことがないのでとりあえずはこの挙動で我慢する他ない。
誰か作ってください。)
== フォント
和文はNoto Serif CJK JPに、欧文はNew Computer Modernに設定される。
このとき、和文フォントは欧文フォントの約0.921倍の大きさにスケールされる。
これにより、欧文が10~DTP~pointのとき和文がちょうど13級になる。
和文ではカーニングを抑止する。
== 行末空白抑止 <行末空白抑止>
改行
しても、
その場所に空白が挿入されることはなくなる。
ただし、欧文の後ろでの改行も同様に消えてなくなるので注意しなければならない。
これは、
```
#set text(spacing: 0%)
```
とすることによって実現している。
この処理はしばしば(特に構文の前後で)通常の欧文のword spaceの挿入処理と干渉し、
本来挿入されるべきword spaceが消えてなくなるという現象が確認されている。
このような場合には` #" " `として明示的に空白を挿入するか、それでもダメであれば
` #text(spacing: 100%) `を局所的に設定する他ない。
== 和文欧文間空白
ソースコード上で直接隣り合ってる和文と欧文のうち、間に和文欧文間空白を入れるべき箇所には
自動でword spaceが挿入される。
それ以外の箇所には手動で挿入する必要がある。
@行末空白抑止 #" "節も参照のこと。
#strike[フォントで何とかならないだろうか。]
== 縦書き
#align(center,
tate(box(width: 32.0675mm, block[
部分的な縦書きを行うことができる。
Alphabetも使える。
justificationが不完全で
禁則処理は崩壊。
連続した約物が調整されない。。。
ページ全体に対して自動で適用することはできない。
残念ながら、改行を含む場合には使い物にならない。
]))
)
= otypjc
日本語向けのレイアウト設定をするモジュール。
好みも入ってる。
otypjpは自動で読み込まれる。
あまり凝った調整はできず、otypjpを使用した時の文書レイアウトの設定例といったところか。
見出し直後に通常の文字の大きさを超えたオブジェクトがある場合、
その場所でページ分割が発生する可能性がある。
= License
#link("https://github.com/tairahikaru/old-typst-japanese")[Web上]で配布している。
/ otypjp.typ, otypjc.typ: #link("https://www.gnu.org/licenses/agpl-3.0.html")[AGPLv3].
/ README.typ, README.pdf: #h(0.001fr)#box(link("https://creativecommons.org/licenses/by-sa/4.0/")[CC BY-SA 4.0]).#h(1fr)
/ scsnowman.typ: #link("https://opensource.org/license/bsd-2-clause/")[BSD 2-Clause].
scsnowman.typはHironobu Yamashita氏による#" "#LaTeX#" "向けの#" "
#link("https://github.com/aminophen/scsnowman")[scsnowmanパッケージ](BSD 2-Clause)を元に制作した。
#import "scsnowman.typ"
#box(height: 56.875mm)[]
#v(-56.875mm)
#v(1fr)
#align(center, scsnowman.scsnowman(
scale: 10,
adjustbaseline: true,
muffler: rgb(100%,0%,0%),
arms: rgb(64.8%,16.5%,16.5%),
snow: rgb(53%,80.8%,92%),
hat: rgb(0%,50%,0%),
buttons: rgb(25.5%,41%,88.4%),
note: rgb(100%,0%,0%),
))
|
https://github.com/Tiggax/famnit_typst_template | https://raw.githubusercontent.com/Tiggax/famnit_typst_template/main/README.md | markdown | MIT No Attribution | # FAMNIT


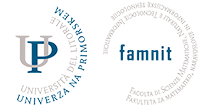
*University of Primorska,*
*Faculty of Mathematics, Natural Sciences and Information Technologies*
---
This is a Typst template for FAMNIT final work.
# Configuration
---
## configuration example
```typst
#import "@preview/sunny-famnit:0.2.0": project
#show project.with(
date: datetime(day: 1, month: 1, year: 2024), // you could also do `datetime.today()`
text_lang: "en" // the language that the thesis is gonna be written in.
author: "your name"
studij: "your course",
mentor: (
name: "his name",
en: ("prepends","postpends"), // you can prepend or postpend any titles
sl: ("predstavki","postavki"),// you can prepend or postpend any titles
),
somentor: none, // if you have a co-mentor write him here the same way as mentor, else you can just remove the line.
work_mentor: none, // if you have a work mentor, the same as above
naslov: "your title in slovene",
title: "your title",
izvleček: [
your abstract in slovene.
],
abstract: [
your abstract
],
ključne_besede: ("Typst", "je", "super!"),
key_words: ("Typst", "is", "Awesome!"),
kratice: (
"Famnit": "Fakulteta za matematiko naravoslovje in informacijske tehnologije",
"PDF": "Portable document format",
),
priloge: (), // you can add attachments as a dict of a title and content like `"name": [content],`
zahvala: [
you can add an acknowlegment.
],
bib_file: bibliography(
"my_references.bib",
style: "ieee",
title: [Bibliography],
),
/* Additional content and their defaults
kraj: "Koper",
*/
)
// Your content goes below.
```
## Abbreviations (kratice)
You can specify Abbreviations at the start as an attribute `kratice` and pass it a dictionary of the abbriviation and it's explanation.
Then you can reference them in text using `@<short name>` to create a link to it.
## Attachments
Some thesis need Attachments that are shown at the end of the file.
To add these attachments add them in your project under `priloge` as a dictionary of the attachment name and its content.
I suggest having a seperate `attachments.typ` file, from where you can reference them in the main project.
## Language
The writing of the thesis can be achieved in two languages; Slovene and English.
They have some differences between them in the way the template is generated, as the thesis needs to be different for each one.
you can specify the language with the `text_lang` attribute.
---
If you have any questions, suggestion or improvements open an issue or a pull request [here](https://github.com/Tiggax/famnit_typst_template)
|
https://github.com/simon-epfl/notes-ba3-simon | https://raw.githubusercontent.com/simon-epfl/notes-ba3-simon/main/comparch/resume.typ | typst | == Tricks
=== Multiplication avec shift et log
Quand on veut calculr $a dot b$, si on dispose du $log_a$ on peut faire $b << log_a$.
=== Charger un mot au milieu
Toutes les adresses doivent être alignées sur la taille des données qu'elles manipulent (donc quand on fait un `lw` ça doit être un multiple de 4). Mais RISCV va modifier le code pour tomber sur le mot juste avant si on utilise un `lw` qui n'est pas un multiple de 4. `lw_ecrit = 4k + r`, donc `lw_corrige = 4k`.
=== Charger un immédiat avec 32 bits
`li` supporte le chargement d'un immediate de 32 bits (tandis que toutes les autres opérations comme `addi`, la constante devant `lw`, etc.) ne supportent que des 12 bits.
=== Convention d'appel
Même si on sait que la fonction `b` que notre fonction `a` va appeler ne modifie pas les saved registers, la fonction appelante doit *TOUJOURS* utiliser et sauvegarder les saved registers
```yasm
addi sp, sp, -12 # mettre à jour le stack pointer (on le fait remonter dans la mémoire)
sw ra, 0(sp)
sw s1 4(sp)
sw s2, 8(sp) # 8... + 4 = 12 bytes libérés utilisés
# blabla
lw ra, 0(sp)
lw s1, 4(sp)
lw s2, 8(sp)
addi sp, sp, 12
```
|
|
https://github.com/galaxia4Eva/galaxia4Eva | https://raw.githubusercontent.com/galaxia4Eva/galaxia4Eva/main/typst/Economist%20Dec%202023/document.typ | typst | #set page(width: 1558pt, height:2048pt, background: image("./images/economist.jpeg"), margin: 0pt)
#align(bottom)[
#grid(
columns:(1fr,1fr,1fr),
rows:(256pt),
[
#align(left)[
#image("./images/Two_orangutani.png")
]
],
[],
[
#align(right)[
#image("./images/One_orangutang.png")
]
]
)
]
|
|
https://github.com/Enter-tainer/typstyle | https://raw.githubusercontent.com/Enter-tainer/typstyle/master/tests/assets/unit/import.typ | typst | Apache License 2.0 | #import "@preview/fletcher:0.4.0" as fletcher: node, edge
|
https://github.com/LuminolT/SHU-Bachelor-Thesis-Typst | https://raw.githubusercontent.com/LuminolT/SHU-Bachelor-Thesis-Typst/main/template/abstract.typ | typst | #import "font.typ" : *
#import "../body/info.typ": *
#import "../body/abstract.typ": *
#pagebreak()
// 中文摘要页
#v(2em)
#align(center)[
#text(font:heiti, size:26pt)[#title]
#v(26pt)
#text(font:heiti, size:18pt)[摘要]
]
#par(
justify: false,
leading: 1.5em,
first-line-indent: 2em)[#text(font: songti, size: 12pt)[#abstract_zh]]
#v(5pt)
#par(first-line-indent: 0em)[
#text(weight: "bold", font: songti, size: 12pt)[
关键词: ]
#text(font: songti, size: 12pt)[#keywords_zh.join(";") ]
]
#pagebreak()
//英文摘要页
#v(2em)
#align(center)[
#text( font: heiti, size: 18pt, "Abstract")
]
#par(justify: false,
leading: 1.5em,
first-line-indent: 2em)[#text(font: songti, size: 12pt)[#abstract_en]]
#v(5pt)
#par(first-line-indent: 0em)[
#text(font: heiti, size: 12pt, weight: "bold")[
Keywords: ]
#text(font: heiti, size: 12pt)[#keywords_en.join("; ") ]
]
|
|
https://github.com/ukihot/igonna | https://raw.githubusercontent.com/ukihot/igonna/main/const.typ | typst | #let me="<NAME>"
#let title="Introduction to Development" |
|
https://github.com/congyu711/matroidslides5min | https://raw.githubusercontent.com/congyu711/matroidslides5min/main/main.typ | typst | MIT License | #import "@preview/touying:0.4.2": *
// #import "@preview/a2c-nums:0.0.1": int-to-cn-ancient-num
#import "@preview/octique:0.1.0": *
#import "@preview/lovelace:0.3.0": *
#import "@preview/ctheorems:1.1.2": *
#show: thmrules.with(qed-symbol: $square$)
#set page(width: 16cm, height: auto, margin: 1.5cm)
#set heading(numbering: "1.1.")
#let theorem = thmbox("theorem", "Theorem", fill: rgb("#eeffee"))
#let corollary = thmplain(
"corollary",
"Corollary",
base: "theorem",
titlefmt: strong
)
#let definition = thmbox("definition", "Definition", inset: (x: 1.2em, top: 1em)).with(numbering: none)
#let example = thmplain("example", "Example").with(numbering: none)
#let proof = thmproof("proof", "Proof")
#let problem = thmbox("problem", "Problem", fill: rgb("#eeffee")).with(numbering: none)
// Functions
#let linkto(url, icon: "link") = link(url, box(baseline: 30%, move(dy: -.15em, octique-inline(icon))))
#let keydown(key) = box(stroke: 2pt, inset: .2em, radius: .2em, baseline: .2em, key)
#let s = themes.university.register(
aspect-ratio: "16-9",
footer-a: self => self.info.author,
footer-c: self => h(1fr) + utils.info-date(self) + h(1fr) + states.slide-counter.display() + h(1fr)
)
#let s = (s.methods.info)(
self: s,
title: [Briefly, what is a Matroid #footnote[#text(weight: "regular")[The title is borrowed from https://www.math.lsu.edu/~oxley/matroid_intro_summ.pdf \ \ ]]],
author: [丛宇],
date: datetime(year: 2024, month: 8, day: 13),
institution: [UESTC],
)
// NO SECTION SLIDES
#(s.methods.touying-new-section-slide = none)
// #let s = (s.methods.datetime-format)(self: s, "[year] 年 [month] 月 [day] 日")
// hack for hiding list markers
#let s = (s.methods.update-cover)(self: s, body => box(scale(x: 0%, body)))
// // numbering
// #let s = (s.methods.numbering)(self: s, "1.")
// // handout mode
// #let s = (s.methods.enable-handout-mode)(self: s)
#let (init, slides, touying-outline, alert) = utils.methods(s)
#show: init
// global styles
#set text(font: ("IBM Plex Serif", "Source Han Serif SC", "Noto Serif CJK SC"), lang: "zh", region: "cn")
#set text(weight: "medium")
#set par(justify: true)
#set raw(lang: "typ")
#set underline(stroke: .05em, offset: .25em)
#show raw: set text(font: ("IBM Plex Mono", "Source Han Sans SC", "Noto Sans CJK SC"))
#show raw.where(block: false): box.with(
fill: luma(240),
inset: (x: .3em, y: 0em),
outset: (x: 0em, y: .3em),
radius: .2em,
)
#show raw.where(block: true): set par(justify: false)
#show strong: alert
#let (slide, empty-slide, title-slide, focus-slide) = utils.slides(s)
#show: slides
= independence structure
== Matroids
#slide[
#set text(weight: "regular",size: 0.8em)
A _matroid_ is an important structure in combinatorial optimization, which generalizes the notion of linear independence in vector spaces.
#definition("Matroid")[
A matroid $M$ is a pair $(E,cal(I))$, where $E$ is a finite set (ground set) and $cal(I)$ is a family of subsets of $E$ (independence sets). The family $cal(I)$ satisfies the following properties:
- $emptyset in cal(I)$
- If $I in cal(I)$ and $J subset.eq I$, then $J in cal(I)$
- If $I, J in cal(I)$ and $|I| < |J|$, then there exists an element $x in J - I$ such that $I union {x} in cal(I)$.
]
Matroid is a blueprint for what independence should mean.
]
== combinatorial optimization
#slide[
combinatorial optimization ≈ optimize over discrete structures
#show: columns.with(2)
#set text(size: 0.8em)
#figure(
numbering: none,
image("images/knapsack.png", width: 40%),
caption: [
#text(size: 0.6em)[The knapsack problem is a classic \ combinatorial optimization problem. \ The goal is finding the most valuable combination of items that can be fit into a knapsack of limited capacity.]
]
)
#colbreak()
#set par(justify: false)
CO problems are often NP-hard.
However, some discrete structures allow efficient optimization algorithms.
#v(2em)
#highlight[Matroid] is such a structure.
]
= from an algebraic perspective
== linearly independent sets
#slide[
#set text(size: 0.6em)
Matrix is just a rectangular array of numbers, e.g.,
#set math.mat(delim: "[")
$
mat(
1, 2, ..., 10;
2, 2, ..., 10;
dots.v, dots.v, dots.down, dots.v;
10, 10, ..., 10;
)
$
set of column vectors
$
mat(
vec(1,2,dots.v,10), ..., vec(10,10,dots.v,10))
$
The family of linearly independent columns forms a matroid.
][
#set text(size:0.6em)
$cal(I)=$ the family of linearly independent columns vectors
- $emptyset in cal(I)$ #text(fill: olive)[(trivially true)]
- If $I in cal(I)$ and $J subset.eq I$, then $J in cal(I)$ #text(fill: olive)[(subsets of linearly independent columns are linearly independent!)]
- If $I, J in cal(I)$ and $|I| < |J|$, then there exists an element $x in J - I$ such that $I union {x} in cal(I)$. #text(fill: olive)[(this is the Steinitz exchange lemma in linear algebra. #linkto("https://en.wikipedia.org/wiki/Steinitz_exchange_lemma"))]
#v(2em)
This matroid is called the _vector matroid_.
]
= from the perspective of graph theory
== Spanning Trees
#slide[
#set text(size: 0.6em)
A _spanning tree_ of a graph $G=(V,E)$ is a subgraph of $G$ and contains all the vertices of $G$ but no cycle.
#figure(
numbering: none,
image("images/spanningtree.png", width: 50%),
caption: [
#text(size: 0.6em)[A graph with 6 vertices and 8 edges.\ The 3 red edges form a cycle. \ The 5 green edges form a spanning tree.]
]
)
][
#set text(size: 0.6em)
$E=$ the set of edges in $G$ \
$cal(I)=$ the family of trees(subgraphs without cycles)
- $emptyset in cal(I)$ #text(fill: olive)[(trivially true)]
- If $I in cal(I)$ and $J subset.eq I$, then $J in cal(I)$ #text(fill: olive)[(subset of a tree is still a tree!)]
- If $I, J in cal(I)$ and $|I| < |J|$, then there exists an element $x in J - I$ such that $I union {x} in cal(I)$. #text(fill: olive)[(try to prove it )]
#v(2em)
This matroid is called the _graphic matroid_.
]
= from the perspective of optimization
== Greedy Algorithm
#slide[
#set text(size: 0.6em)
#problem("optimization over matroids")[
Given a matroid $M=(E,cal(I))$ and a weight function $w:E arrow.r R^+$, find a maximum-weight independent set.
]
#figure(
numbering: none,
pseudocode-list(hooks: .5em,booktabs: true, title: smallcaps[Max-Weighted Independent Set])[
+ let $E={e_1,...,e_n}$ such that $w(e_1) >= w(e_2) >= ... >= w(e_n)$
+ $x arrow.l emptyset$
+ *for* $i=1$ to $n$ do
+ *if* $X union {e_i} in cal(I)$ *then*
+ $X arrow.l X union {e_i}$
+ *return* $X$
]
)<alg>
- For vector matroids, the greedy algorithm is conceptually the same as Gaussian elimination.
- For graphic matroids, the greedy algorithm is the same as Kruskal's algorithm.
] |
https://github.com/barrel111/readings | https://raw.githubusercontent.com/barrel111/readings/main/classes/cs6840/notes.typ | typst | #import "@local/preamble:0.1.0": *
#import "@preview/lovelace:0.2.0": *
#show: project.with(
course: "CS6840",
sem: "FA24",
title: "Algorithmic Game Theory",
subtitle: "Notes",
authors: (
"<NAME>",
),
)
#show: setup-lovelace
= Introduction
=
=
#pagebreak()
= The Multiplicative Weights Algorithm
\
We consider the following model.
#algorithm(
caption: [Binary Labeling with Expert Advice],
pseudocode-list[
- Time proceeds in rounds $(1, 2, dots, T)$
- There are $K$ experts $(1, dots, K)$
- There are $2$ labels $(0, 1)$
- Each round proceeds as follows
- Each expert recommends a label
- Algorithm $ALG$ guesses label after seeing recommendations
- Correct label revealed
- *Goal*: Minimize number of mistakes made by $ALG$.
]
)
We consider a few candidates for $ALG$.
First, consider the case where there exists a perfect expert.
#algorithm(
caption: [`MAJORITY`],
pseudocode-list[
- *Initialize* $w_i = 1$ for all $i in [k]$
- *Set* an expert's weight to $0$ whenever it makes a mistake
- *Predict* label $0$ or $1$ according to a weighted majority vote of experts.
]
)
#lemma[`MAJORITY` makes $<= floor(log_2(K))$ mistakes. ]
#lemma[Every deterministic algorithm makes $>= floor(log_2(K))$ mistakes on some input.]
#lemma[For any randomized algorithm, $EE(ALG) <= 1/2 floor(log_2(K))$.]
Now, we move on to the case where there is no guarantee that a perfect expert exists. We want to make not-too-many mistakes than the best expert.
#algorithm(
caption: [`WEIGHTED MAJORITY`],
pseudocode-list[
- *Initialize* $w_i = 1$ for all $i in [k]$
- *Whenever* an expert makes a mistake, multiply $w_i$ by $1 - epsilon$
- *Predict* labels by taking a weighted majority vote.
]
)
If $W_(t - 1)$ and $W_t$ are the combined weight after $t -1$ and $t$ rounds, respectively, then $ W_t <= (1 - epsilon/2) W_(t - 1). $
If there exists an expert making only $m$ mistakes then, $ (1 - epsilon)^m < W_T < (1 - epsilon/2)^(ALG) dot W_0 = (1 - epsilon/2)^(ALG). $
Then, $ m ln(1 - epsilon) < ln K + ALG ln (1 - epsilon/2) \ => - ALG ln (1 - epsilon/2) < ln K - m ln(1 - epsilon) \ => ALG < - (ln K)/(ln(1 - epsilon/2)) + m (ln(1 - epsilon))/(ln (1 - epsilon/2)) $
For $0 < epsilon < 1/2$, $epsilon < - ln (1 - epsilon) < epsilon + epsilon^2$. Thus, $ < (2 ln K)/epsilon + (2 + epsilon) m. $
This $epsilon$ is also known as the _learning rate_. Note that the factor of $2$ in the term $2m$ is unavoidable for deterministic algorithms.
#lemma[For every deterministic $ALG$, we have $ALG >= 2m$ on some input.]
= Randomized Multiplicative Weights (Hedge)
In this lecture, we introduce a randomized algorithm that saves the factor of $2$ from the previous lecture. The setting we consider is more general than the binary labelling with expert advice model we workedwith last time.
#algorithm(
caption: [`Model`],
pseudocode-list[
- $K$ experts
- At each time $t$:
- $ALG$ selects a probability distribution over $[K]$, $p_t$,
- `ADV` selects loss vector, $ell_t in [0, 1]^K$.
- $p_t, ell_t$ may depend on ${(p_s, ell_s) bar s = 1, dots, t- 1}$
]
)
Loss of expert $i$ is $ell_t (dot.c)$. Algorithm loss is $ inner(ell_t, p_t) = sum_{i = 1}^K ell_t(i) p_t(i)$. We also define $L_t = sum_( s = 1 )^t ell_s$. Then, our algorithm `HEDGE`$(epsilon)$ is specified by the following scheme for $t = 1, 2, dots, T$:
$ w_t(i) &= (1- epsilon)^(L_(t- 1)(i)) \ W_t &= sum_{i = 1}^K w_t (i) \ p_t (i) &= (w_t (i))/W_t $
Now, we move on to the analysis of `HEDGE`. Consider,
$ w_(t + 1) (i) = (1 - epsilon)^(L_t (i)) &= (1 - epsilon)^(L_(t - 1)(i) + ell_t(i)) \ &= (1 - epsilon)^(ell_t (i)) dot w_t (i) $
#lemma[For $x in [0, 1]$, $(1 - epsilon)^x <= 1 - epsilon x$.]
So, $ &<= [1 - epsilon ell_t (i)] dot w_t (i). $
Next, $ W_(t + 1) = sum_(i = 1)^K w_(t + 1) (i) &<= sum_(i = 1)^K [1 - epsilon ell_t (i)] w_t(i) \ &= sum_(i = 1)^K w_t(i) - epsilon sum_( i = 1 ) ell_t (i) p_t (i) W \ &= W_t [1 - epsilon inner(ell_t, p_t)] $
Then,
$ ln W_(t + 1) &<= ln W_t + ln (1 - epsilon inner(ell_t, p_t)) \ &<= ln W_t - epsilon inner(ell_t, p_t) $
Inductively,
$ ln W_(T + 1) <= ln W_0 - epsilon sum_(t = 1)^T inner(ell_t, p_t) $
If $i^star$ is the best expert, that is $L_T(i^star) = min_(j in [k]) L_T(i)$, then $ W_(T + 1) = sum_(i = 1)^K (1 - epsilon)^(L_T (i)) > (1 - epsilon)^(L_T (i^star)) \ => ln W_(T + 1) > ln (1 - epsilon) dot L_T (i^star). $
Thus,
$ ln (1 - epsilon) dot L_T (i^star) &< ln K - epsilon sum_(t = 1)^T inner(ell_t, p_T) \ ==> sum_(t = 1)^T inner(ell_t, p_t) &<= (ln K)/epsilon + (- ln (1 - epsilon))/epsilon dot L_T (i^star) \ &< (ln K)/epsilon + (epsilon + epsilon^2)/epsilon dot L_T (i^star) \ &= (ln K)/epsilon + (1 + epsilon) L_T (i^star). $
How do we choose $epsilon$? One response would be to minimize _regret_.
#definition[$ cal(R)^ALG (T) = underbrace(sum_(t = 1)^T inner(ell_t, p_T^ALG), ALG "loss") - underbrace(L_T (i^star), "best expert's loss") $]
For `HEDGE`,
$ R^mono("HEDGE") (T) &< (ln K)/epsilon + epsilon L_T (i^star) \ &<= (ln K)/epsilon + epsilon T $
Optimizing the right hand side, we obtain $epsilon = sqrt((2 ln K)/T)$ which gives us
$ R^mono("HEDGE")(T) < sqrt(2 (ln K) T). $
Shouldn't hope to do better than $sqrt(T)$.
Another way of choosing $epsilon$ would be the _doubling trick_. At time $t$, we don't yet know $L_T (i^star)$ but we do know $ min_(i in [K]) {L_(t - 1) (i)} $ Start by assuming $L_T (i^star)$ will be $<= 1$.
Run epochs $0, 1, 2, dots$. In epoch $j$, we assume $L_T (i^star)$ will be $<= 2^j$ and set $epsilon$ to minimize $ (ln K)/epsilon + epsilon 2^j ==> epsilon = sqrt((2 ln K)/2^j). $ Then epoch $j$ ends with $min_(i) {L_(t - 1) (i) } > 2^j$. Then re-initialize `HEDGE` and begin epoch $j + 1$. Then,
$ "Regret"("epoch" j) <= (ln K)/epsilon_j + epsilon_j 2^j = sqrt(2 (ln K) 2^j) $
If we end in epoch $J$,
$ "Regret" &<= sum_(j = 0)^J sqrt(2 (ln K) 2^j) \ &= sqrt(2 ln K) sum_(j = 0)^J 2^(j \/ 2) = O(sqrt(2 (ln K) 2^J)) = O(sqrt(2 (ln K) L_T (i^star))) $
#remark[In the past two lecture, we considered learning in a setting with $K$ experts with associated loss $ell_t (i)$ at time $t$ for expert $i$. In the context of games, experts correspond to _strategies_ and loss corresponds to _cost_ or _utility_. That is, loss corresponds to the cost you undertake when you choose the given strategy, $ell_t (i) tilde c_i (s_i, s_(-i)^t) = ell_t (s_i)$.]
= Auctions
== Single Item Auctions
Consider a seller with a single item. There are $n$ players or bidders. We assume that each bidder $i$ has a nonnegative _valuation_ $v_i$ that is _private_, meaning it is unknown to the seller and other bidders. The auction proceeds in three steps
+ Ask all players for a bid $b_i$
+ Select winner. For now, we select $"argmax"_i b_i$.
+ Announce required payment. There may be a few choices for what this payment ought to be
- _first price_: Set _price_ = $max_i b_i$.
- _second price_: Suppose $i^star = "argmax"_i b_i$. Then, _price_= $max_(i != i^star) b_i$.
- _all pay_: Everyone pays their bid.
We adopt a _quasilinear utility model_, wherein $ u(b_i) = cases(0 &"if" b_i "loses,", p - v_i #h(16pt) &"if" b_i "wins at price" p.) $
#definition[A _dominant strategy_ is a strategy that is guaranteed to maximize a bidder's utility no matter what the other bidders do.]
#prop[In second-price auctions, the dominant strategy is to use $b_i = v_i$.]
#proof[ Consider a bidder $b_i$. Let $B = max_(j != i) b_j$. There are two cases to consider
- if $B > v_i$ then the maximum utility $b_i$ can receive is $0$, which is acquired by truthfully bidding $b_i = v_i$,
- if $B < v_i$ then the maximum utility $b_i$ can receive is $v_i - B$ which is acquired by truthfully bidding $b_i = v_i$.]
For first price, analysis depends on the level of knowledge that bidders have.
All-price auctions are more interesting to consider. Consider the following example.
#example[There are two players with valuations $v_1 = v_2 = 1$. All players are also aware of this. There is no Pure Strategy Nash Equilibrium here. However, we propose a Mixed Strategy Nash Equilibrium where we pick $b_i in [0, 1]$ uniformly at random. Consider the vaue for player $1$ under the pure strategy $b_i = x$. The utility of this strategy is $-x + x = 0$. However, utility for randomization is also $0$.]
#example[Consider two players with valuations $v_1 = 1, v_2 = 2$ with both players aware of the valuations. Then we have a Mixed Nash Equilibrium at
$ b_2 in [0, 1] "uniformly random," $
$ b_1 = cases(0 "prob 1/2,", [0, 1] "unif. prob 1/2.") $]
= Learning in 2-Person Games
|
|
https://github.com/fenjalien/cirCeTZ | https://raw.githubusercontent.com/fenjalien/cirCeTZ/main/utils.typ | typst | Apache License 2.0 | #import "../typst-canvas/vector.typ"
#import "../typst-canvas/draw.typ": anchor
#let vector-dist(v1, v2) = {
return calc.abs(
vector.len(
vector.sub(
v2,
v1
)
)
)
}
#let get-angle(v1, v2, debug: false) = {
// panic(v1, v2)
let (x,y,z) = vector.sub(v2, v1)
return calc.atan2(x,-y)
// return -panic(if v2.at(1) < v1.at(1) {-1} else {1} * vector.angle(vector.sub(v2, v1), (0,0,0), (1,0,0)))
// return -if v2.at(1) < v1.at(1) {-1} else {1} * vector.angle(vector.sub(v2, v1), (0,0,0), (1,0,0))
}
#let vector-rotate(v, angle) = {
let (x, y, z) = v
// panic(angle)
(
x * calc.cos(angle) - y * calc.sin(angle),
x * calc.sin(angle) + y * calc.cos(angle),
z
)
}
#let defer-rotation(v, v1, v2) = {
(
(v, v1, v2) => {
// panic(v)
// panic(v1, v2)
vector-rotate(v, -get-angle(v1, v2))
},
v,
v1,
v2
)
}
// #let reverse-transform(v) = {
// (
// (start, center, v) =>
// )
// }
#let anchors(anchors) = {
for (k, v) in anchors {
anchor(k, v)
}
} |
https://github.com/jgm/typst-hs | https://raw.githubusercontent.com/jgm/typst-hs/main/test/typ/math/underover-02.typ | typst | Other | // Test brackets.
$ underbracket([1, 2/3], "relevant stuff")
arrow.l.r.double.long
overbracket([4/5,6], "irrelevant stuff") $
|
https://github.com/typst/packages | https://raw.githubusercontent.com/typst/packages/main/packages/preview/unichar/0.1.0/ucd/block-31F0.typ | typst | Apache License 2.0 | #let data = (
("KATAKANA LETTER SMALL KU", "Lo", 0),
("KATAKANA LETTER SMALL SI", "Lo", 0),
("KATAKANA LETTER SMALL SU", "Lo", 0),
("KATAKANA LETTER SMALL TO", "Lo", 0),
("KATAKANA LETTER SMALL NU", "Lo", 0),
("KATAKANA LETTER SMALL HA", "Lo", 0),
("KATAKANA LETTER SMALL HI", "Lo", 0),
("KATAKANA LETTER SMALL HU", "Lo", 0),
("KATAKANA LETTER SMALL HE", "Lo", 0),
("KATAKANA LETTER SMALL HO", "Lo", 0),
("KATAKANA LETTER SMALL MU", "Lo", 0),
("KATAKANA LETTER SMALL RA", "Lo", 0),
("KATAKANA LETTER SMALL RI", "Lo", 0),
("KATAKANA LETTER SMALL RU", "Lo", 0),
("KATAKANA LETTER SMALL RE", "Lo", 0),
("KATAKANA LETTER SMALL RO", "Lo", 0),
)
|
https://github.com/ooliver1/a-level-project | https://raw.githubusercontent.com/ooliver1/a-level-project/master/writeup/palettes/xcolor.typ | typst | /* The colors provided by xcolor
*
* Source: https://en.wikibooks.org/wiki/LaTeX/Colors
* Accessed: 2023-03-31
*/
#let xcolor = (
apricot : rgb("#fbb982"),
aquamarine : rgb("#00b5be"),
bittersweet : rgb("#c04f17"),
black : rgb("#221e1f"),
blue : rgb("#2d2f92"),
blue-green : rgb("#00b3b8"),
blue-violet : rgb("#473992"),
brick-red : rgb("#b6321c"),
brown : rgb("#792500"),
burnt-orange : rgb("#f7921d"),
cadet-blue : rgb("#74729a"),
carnation-pink : rgb("#f282b4"),
cerulean : rgb("#00a2e3"),
cornflower-blue : rgb("#41b0e4"),
cyan : rgb("#00aeef"),
dandelion : rgb("#fdbc42"),
dark-orchid : rgb("#a4538a"),
emerald : rgb("#00a99d"),
forest-green : rgb("#009b55"),
fuchsia : rgb("#8c368c"),
goldenrod : rgb("#ffdf42"),
gray : rgb("#949698"),
green : rgb("#00a64f"),
green-yellow : rgb("#dfe674"),
jungle-green : rgb("#00a99a"),
lavender : rgb("#f49ec4"),
lime-green : rgb("#8dc73e"),
magenta : rgb("#ec008c"),
mahogany : rgb("#a9341f"),
maroon : rgb("#af3235"),
melon : rgb("#f89e7b"),
midnight-blue : rgb("#006795"),
mulberry : rgb("#a93c93"),
navy-blue : rgb("#006eb8"),
olive-green : rgb("#3c8031"),
orange : rgb("#f58137"),
orange-red : rgb("#ed135a"),
orchid : rgb("#af72b0"),
peach : rgb("#f7965a"),
periwinkle : rgb("#7977b8"),
pine-green : rgb("#008b72"),
plum : rgb("#92268f"),
process-blue : rgb("#00b0f0"),
purple : rgb("#99479b"),
raw-sienna : rgb("#974006"),
red : rgb("#ed1b23"),
red-orange : rgb("#f26035"),
red-violet : rgb("#a1246b"),
rhodamine : rgb("#ef559f"),
royal-blue : rgb("#0071bc"),
royal-purple : rgb("#613f99"),
rubine-red : rgb("#ed017d"),
salmon : rgb("#f69289"),
sea-green : rgb("#3fbc9d"),
sepia : rgb("#671800"),
sky-blue : rgb("#46c5dd"),
spring-green : rgb("#c6dc67"),
tan : rgb("#da9d76"),
teal-blue : rgb("#00aeb3"),
thistle : rgb("#d883b7"),
turquoise : rgb("#00b4ce"),
violet : rgb("#58429b"),
violet-red : rgb("#ef58a0"),
white : rgb("#ffffff"),
wild-strawberry : rgb("#ee2967"),
yellow : rgb("#fff200"),
yellow-green : rgb("#98cc70"),
yellow-orange : rgb("#faa21a"),
)
|
|
https://github.com/MrToWy/Bachelorarbeit | https://raw.githubusercontent.com/MrToWy/Bachelorarbeit/master/Code/getValue.typ | typst | #import("../Template/customFunctions.typ"): *
#codly(
highlights:(
(line:13, label: <getValueFromPath>),
(line:22, label: <getNestedProperty>),
(line:26, fill:green, label: <reduce>),
(line:28, fill:red, label: <detectArray>),
(line:32, fill:blue, label: <ignoreVar>),
)
)
```ts
private async getValue(module: any, lang: string, paths: PdfStructureItemPathIncludingField[]): Promise<string> {
let result = "";
for (const path of paths) {
result += await this.getValueFromPath(module, lang, path.field.path ?? "") + " " + path.field.suffix;
}
return this.formatForLatex(result, lang);
}
private async getValueFromPath(module: any, lang: string, path: string): Promise<any> {
if (path in this.specialPaths) {
const result = this.specialPaths[path](module, lang);
if (result instanceof Promise) {
return await result;
}
return result;
} else {
return this.getNestedProperty(module, path);
}
}
private getNestedProperty(obj: any, path: string): any {
const pathSegments = path.split(".");
// Use reduce to traverse the object structure
return pathSegments.reduce((currentValue, segment) => {
// Check if the part matches the pattern "arrayName[index]"
const match = segment.match(/^(\w+)\[(\d+)]$/);
if (match) {
// match consists of the full match, the array name and the index
const [_, arrayName, index] = match;
return currentValue && currentValue[arrayName] && currentValue[arrayName][parseInt(index)];
}
return currentValue && currentValue[segment];
}, obj);
}
private getSubmodules(module: any, lang: string): string {
if (module.subModules && module.subModules.length > 0) {
return module.subModules.map((subModule: any) => {
return `${subModule.abbreviation}$\\quad$${subModule.translations[activeTranslationIndex].name}, ${this.translations[lang]?.submoduleRequired.true}`;
}).join(" \\\\ ");
} else {
return "-";
}
}
``` |
|
https://github.com/Kasci/LiturgicalBooks | https://raw.githubusercontent.com/Kasci/LiturgicalBooks/master/CU/minea/1_generated/00_all/1_minea.typ | typst | #include "03_november.typ"
#include "04_december.typ"
|
|
https://github.com/rabotaem-incorporated/algebra-conspect-1course | https://raw.githubusercontent.com/rabotaem-incorporated/algebra-conspect-1course/master/sections/06-field-theory/05-finite-fields.typ | typst | Other | #import "../../utils/core.typ": *
== Классификация конечных полей
#ticket[Эндоморфизм возведения в степень $p$ поля характеристики $p$]
#pr[
Пусть $char F = p in PP_(>0)$. Существует эндоморфизм $Phi$, который ведет себя следующим образом:
$
F &limits(-->)^(Phi) F \
x &maps x^p.
$
Такой эндоморфизм называется _эндоморфизмом Фробениуса_.
]
#proof[
$
Phi(x y) = (x y)^p = x^p y^p = Phi(x) Phi(y), \
Phi(1) = 1^p = 1, \
Phi(x + y) = x^p + y^p + sum_(i=1)^(p-1) underbrace(C_p^i x^i y^(p-i), dots.v space p) = x^p + y^p = Phi(x) + Phi(y).
$
]
#notice[
Если $F$ --- конечное, то $Phi$ --- автоморфизм.
]
#ticket[Возможные порядки конечного поля. Существование поля из $p^n$ элементов]
#pr[
Пусть $F$ --- поле, $abs(F) = q < oo$. Тогда $forall x in F$, $x^q = x$.
]
#proof[
Очевидно при $x = 0$. Если $x != 0$, то $x in F^*$.
$
F^* - ["циклическая"] "группа порядка" q - 1 ==> x^(q-1) = 1 ==> x^q = x.
$
]
#th[
Пусть $q = p^n$. Тогда поле из $q$ элементов существует.
]
#proof[
$F$ --- поле из $p$ элементов. $L fg F$ --- поле разложения $x^q - x$.
Посмотрим на производную: $(x^q - x)' = q x^(q-1) - 1 = -1 ==>$ у $x^q - x$ нет кратных корней.
$a_1, ..., a_q$ --- все корни $x^q - x$, $K = {a_1, ..., a_q}$.
Оказывается, что $K$ --- подполе в $L$, потому что:
$
a, b in K space (a+b)^q - (a+b) = a^q + b^q - a - b = a + b - a - b = 0\
(a b)^q - a b = a^q b^q - a b = a b - a b = 0\
(-a)^q = (-1)^q a^q = underbrace((-1), #place[даже при $p = 2$]) a = -a\
1^q = 1\
$
]
#ticket[Единственность поля из $p^n$ элементов]
#lemma[
Пусть $L fg K$ --- конечное расширение конечного поля. Тогда такое расширение --- простое.
]
#proof[
$L^*$ --- циклическая, то есть $L^* = gen(theta)$. $K(theta) = L$.
]
#th[
Пусть $K_1$ и $K_2$ --- поля и $abs(K_1) = abs(K_2) = p^n$. Тогда $K_1 iso K_2$.
]
#proof[
По лемме, $K_1$ --- простое расширение. $K_1 = FF_p (theta_1)$. Пусть $g = Irr_FF_p theta_1$. $deg g = n$, так как $[K_1 : FF_p] = n$. $theta_1$ --- корень $x^p^n-x$, значит $g divides (x^p^n - x)$.
В $K_2$ все элементы --- корни $x^p^n - x$, значит у него есть $p^n$ корней. Значит $x^p^n - x$ раскадывается на линейные множители в $K_2$. Получается, что $g$ раскладывается на линейные множители в $K_2$. Поэтому $exists theta_2 in K_2: g(theta_2) = 0 ==> [FF_p (theta_2) : FF_p] = n = [K_2 : FF_p] ==> FF_p (theta_2) = K_2$.
Теперь заметим, что если $K_1 = FF_p (theta_1), K_2 = FF_p (theta_2)$ и $Irr_(FF_p (theta_1)) = Irr_(FF_p (theta_2))$, то $K_2 iso K_1$.
]
#denote[
$FF_q$ --- поле из $q$ элементов.
]
#th[
+ Пусть $K$ --- подполе $FF_(p^n)$. Тогда $abs(K) = p^m$, $m divides n$
+ Пусть $m divides n$. Тогда в $FF_(p^n)$ есть единственное подполе из $p^m$ элементов
]
#proof[
+ $char K = p ==> abs(K) = p^m$ для некоторого $m in NN$\
$[FF_(p^n) : K] = d ==> underbrace(abs(FF_(p^n)), p^n) = underbrace(abs(K)^d, (p^m)^d), space n = m d$
+ #[
"Существование": $m divides n ==> (p^m - 1) divides (p^n - 1) ==> (x^(p^m - 1) - 1) divides (x^(p^n - 1) - 1) ==> (x^(p^m) - x) divides (x^(p^n) - x) $
$x^(p^n) - x$ расскладывается на линейные в $FF_(p^n) ==> x^(p^m)- x$ тоже расскладывается на линейные в $FF_(p^n)$
Очевидно, корни разложения: $x_1, ..., x_(p^m)$, ${x_1, ..., x_(p^m)}$ --- искомое подполе.
"Единственность": Пусть $F_1$ и $F_2$ --- подполя $FF_(p^n)$ из $p^m$ элементов
$ forall x in F_1 union F_2 : x^(p^m) - x = 0 ==> abs(F_1 union F_2) <= p^m ==> F_1 = F_2$
]
]
#example[
#import "../../packages/commute.typ": *
#align(center)[#commutative-diagram(
node((0, 1), $FF_4096$),
node((1, 0), $FF_64$),
node((1, 2), $FF_16$),
node((2, 0), $FF_8$),
node((2, 2), $FF_4$),
node((3, 1), $FF_2$),
arr((0, 1), (1, 0), $$),
arr((0, 1), (1, 2), $$),
arr((1, 0), (2, 0), $$),
arr((1, 0), (2, 2), $$),
arr((1, 2), (2, 2), $$),
arr((2, 0), (3, 1), $$),
arr((2, 2), (3, 1), $$)
)]
]
== Автоморфизмы конечных полей. (без доказательств)
$
abs(A u t(FF_(p^n))) = n \
A u t(FF_(p^n)) = gen(Phi)
$
$
Phi: FF_(p^n) &--> FF_(p^n) \
x &maps x^p \
$
$
[L : K] = n ==> \
abs(underbrace(A u t(L fg K), {sigma in A u t L})) <= n
$
|
https://github.com/dismint/docmint | https://raw.githubusercontent.com/dismint/docmint/main/multicore/pset4.typ | typst | #import "template.typ": *
#show: template.with(
title: "6.5081 PSET 4",
subtitle: "<NAME>",
pset: true
)
= Problem 1
== (a)
*Consensus Number*: $infinity$
Recall that using the regular FIFO queue raised problems for any $n > 2$ consensus number, as there was no possible combination of operations at the critical state that could guarantee a consensus. However, with this new `peek()` operation, we can now guarantee a consensus for any $n$. I will work under the assumption that `peek()` is atomic.
I propose the following algorithm:
```java
public class PeekQueueConsensus<T> extends ConsensusProtocol<T> {
PeekQueue pq;
public QueueConsensus() {
// initialize queue
pk = new PeekQueue();
}
public T decide(T Value) {
propose(value);
int i = ThreadID.get();
// propose ourself as the candidate
pq.enq(i);
// see who the actual first place is
int winner = pq.peek();
return proposed[winner];
}
}
```
The idea for this algorithm is that we will propose ourselves as the candidate, adding our ID to the `PeekQueue`. The advantage with this algorithm that a regular `Queue` does not have is that we have the ability to `peek()`, which does not modify the state of the queue. This fixes the main issue with the regular `Queue` algorithm, which is that threads past the first two cannot deduce who the winner is. There will be a first element in the queue, and every single thread can `peek()` to see who that winner was. Thus with this algorithm, we can support a consensus for any number of threads.
== (b)
*Consensus Number*: $2$
First I will show that the consensus number is at least $2$ for a `Stack`. Consider the following algorithm:
```java
public class StackConsensus<T> extends ConsensusProtocol<T> {
private static final int WIN = 0; // first thread
private static final int LOSE = 1; // second thread
Stack stack;
// initialize stack with two items
public StackConsensus() {
stack = new Stack();
stack.push(LOSE);
stack.push(WIN); // WIN is at the top of the stack
}
// figure out which thread was first
public T decide(T Value) {
propose(value);
int status = stack.pop();
int i = ThreadID.get();
if (status == WIN) {
return proposed[i];
} else {
return proposed[1-i];
}
}
}
```
This algorithm is almost the exact same as the simple `Queue` consensus implementation, except the elements are pushed in reverse order since it is a stack.
Now I will show that the consensus number is at most $2$. Consider an exhaustive list of all possibilities at the critical state, where we have threads `a,b,c` and thread `a` moves the protocol to a 0-valent state and thread `b` moves the protocol to a 1-valent state:
+ `push` and `push` - suppose `a` and `b` `push()` in that order. Then `a` and `b` both `pop()` the stack. The stack therefore returns to the original state, and there is no difference to the ordering where `b` went first. Thus for any threads that go after the first two, they are supposed to deduce somehow that either `a` or `b` went first, but they cannot do so.
+ `push` and `pop` - Suppose whichever thread goes first does a `push()` and the next thread does a `pop()`. In this case, the stack will be empty, and the next thread will not be able to deduce who went first. If the order is reversed, regardless of whether the stack starts out empty or not, the final state will be the same, except the top element of the stack, which will either be the result of `a` or `b` doing a `push()`. In both cases, there is another analogous ordering that thread `c` can be confused by. For example, if `a` does a `pop()` and `b` does a `push()`, `c` should deduce that it is `a`-valent, since `a` went first. However, this is functionally no different from the case where `b` does a `push()` by itself, in which case the system is `b`-valent.
+ `pop` and `pop` - Suppose `a` and `b` both `pop()`. There is no way for any thread that comes after them to observe what order they went in, since the stack will contain the same elements, as the `pop()` operations remove the same elements (if they exist).
== (c)
*Consensus Number*: $2$
The consensus number must be at least $2$, since we have all the operations from *(b)*. However, we now have an additional possibility from `peek()` that must be considered in the critical state. I will show an exhaustive list of all possibilities including `peek()` that can happen at the critical state:
+ `peek` and `peek` - Since `peeking` does not change the state of the system, there is no way for any thread that goes after to deduce who went first.
+ `peek` and `pop` - Because `peek()` does not change the state of the system, the stack will look the same after both operations - that is, it will be missing the top element, if there exists one. There is therefore no way for any thread that goes after to deduce who went first.
+ `peek` and `push` - If `a` does a `peek()` and `b` does a `push()`, the stack will contain the same elements, except the top element will be the result of `b` doing a `push()`. If the order is reversed, the stack will contain the same elements, except the top element will be the result of `a` doing a `peek()`. There is no way for any thread that goes after to deduce who went first, as the stack looks exactly the same for some thread `c`
= Problem 2
== (a)
This question can be reduced to a consensus problem using atomic registers. We wish to show that it is possible for a consensus number of $2$. However, we know from class that atomic registers have a consensus number of $1$. Each line can be thought of as a register that contains the integral value of the text in that line. Thus, there is no way that Alice and Bob can exactly coordinate their time under the wait-free condition, as that would require a consensus number of at least $2$, which we know atomic registers do not have.
== (b)
Yes, there is a way for them to reach an approximate closeness in a finite amount of time. We can give "ownership" of the first line to Alice, and "ownership" of the second line to Bob. The protocol then proceeds as follows for either Alice or Bob:
+ Write your time to your register.
+ Read the other person's register. If it is empty, return your time as the decided time.
+ If the other person's register is not empty, take the average of the two times and write that into your register.
+ Repeat *(3)* until you are within the desired $epsilon$
Because the two move toward each other by taking the average, they can only ever get closer to each other, and will never pass by each other. We can always reach an approximation in a finite number of time steps relative to $epsilon$
= Problem 3
== (a)
=== Consistent
Assume for the next sections that we have two threads `a` and `b`
Suppose that `a` sees it is "far behind" and returns `b`. Let us show that `b` must see it is "far ahead" and return itself. At the moment `a` returns, it has a smaller value than `b`. Let us suppose that `b` somehow thought `a` was ahead of it, and returned. If this was the case, then `b` would have a smaller value than `a` from that point onward, since both speeds are positive, and `b` has returned. However, the same logic applies for `a`. Essentially, the thread that returns it is behind first will forever be behind, and it is guaranteed that the other thread has not returned yet, and will eventually see it is ahead. Otherwise it would not have been possible for this thread to return it is less, since the other thread would have returned first.
Suppose that `a` sees it is "far ahead" and returns itself. Let us show that `b` must see it is "far behind" and returns `a`. `a` can only make this claim if it is at least one full loop iteration ahead of `b`. If `b` were to have already returned, it could not have said that it was ahead of `a`. If this was the case, `a` would have seen that `b` returned, since `b` would only have done this if it had a one loop grace period on `a`. Thus, it must be the case that `b` has not returned yet, and since `a` has at least a one loop grace period on `b`, `b` must return `a`.
=== Valid
We only return a proposed value, and the only proposed values that are set are at the beginning of the function by two threads. There are no further modifications to the data structure, meaning we must return one of the two values that were set prior.
== (b)
If the 1-speed thread runs exactly three times faster than the 3-speed thread, then it is impossible for the 1-speed thread to ever be more than 3 ahead of the 3-speed thread. Conversely, the 3-speed thread will never catch up to the 1-speed thread. This will result in the two threads racing infinitely, and thus it cannot be wait free.
= Problem 4
== (a)
Consensus number: $2$
Recall that in an atomic register, this type of class of objects ran into the issue that issuing two writes would always favor the thread that went first. That is, at a critical section, there would be no way for the second thread to deduce who truly went first. This however, is not the case for both of these functions, as they both use the old value in the computation for the new one. First, I will show that the consensus number is at least $2$:
For the `FetchAndMultiply` class, consider the following algorithm. Two threads use a shared `FetchAndMultiply` object, with the initial value being $1$. Then suppose thread `a` calls `FetchAndMultiply(2)`, and thread `b` calls `FetchAndMultiply(3)`. If they see these exact values then they were the first to go, otherwise, they were the second to go, and should return the other element.
For the `FetchAndSquare` class, consider the following algorithm. Two threads use a shared `FetchAndSquare` object, with the initial value being $2$. Then suppose thread `a` calls `FetchAndSquare()`, and thread `b` calls `FetchAndSquare()`. If they see $4$, then they were the first to go, and otherwise, they will see $8$, meaning they should return the other element.
Now I will show that the consensus number is at most $2$:
There is only one combination of operations to consider for both, which is having two of the same calls. In both cases, it is impossible for a third thread `c` to observe any difference, as multiplication and squaring are both commutative operations. It would be impossible for `c` to deduce who went first in this one exhaustive case, and thus the greatest that the consensus number can be is $2$.
== (b)
= Problem 6
They would both cease working as both implementations contain a prerequisite check to make sure the current node was not previously added to the list. We could end up in a scenario where the node was not considered part of the list due to the fact that sentinel number is 0, and does not behave properly at the check each of the implementations have. Thus, this could lead to a catastrophic failure later in the algorithm with this assumption.
= Problem 7
Suppose that other edit happen to a thread's head while they are processing some other function. If we assign this value locally instead of consulting what happened in the rest of the system, we might miss out on critical updates. If this were to happen, the control flow might be altered, and a thread might be confused on where the head as supposed to be. This would lead to a nonlinearizable construction, as the head would not be consistent across all threads. Thus, it would become impossible to get a consistent sequence of operations, and thus the construction would not work anymore.
= Problem 9
Both code snippets were adapted almost verbatim from the textbook sections as linked.
*`CLHLock.java`*
```java
import java.util.concurrent.atomic.*;
public class CLHLock implements Lock {
volatile AtomicReference<QNode> tail = new AtomicReference<QNode>(new QNode());
ThreadLocal<QNode> myPred;
ThreadLocal<QNode> myNode;
public CLHLock() {
// set the initial values for all fields
tail = new AtomicReference<QNode>(new QNode());
myNode = new ThreadLocal<QNode>() {
protected QNode initialValue() {
return new QNode();
}
};
myPred = new ThreadLocal<QNode>() {
protected QNode initialValue() {
return null;
}
};
}
public void lock() {
QNode qnode = myNode.get();
qnode.locked = true;
// set up the chain of nodes
QNode pred = tail.getAndSet(qnode);
myPred.set(pred);
// spin until our predecessor is done
while (pred.locked) {}
}
public void unlock() {
QNode qnode = myNode.get();
// im no longer busy!
qnode.locked = false;
// move to new node
myNode.set(myPred.get());
}
class QNode {
boolean locked = false;
}
}
```
*`MCSLock.java`*
```java
import java.util.concurrent.atomic.*;
public class MCSLock implements Lock {
AtomicReference<QNode> tail;
ThreadLocal<QNode> myNode;
public MCSLock() {
// init all fields
tail = new AtomicReference<QNode>(null);
myNode = new ThreadLocal<QNode>() {
protected QNode initialValue() {
return new QNode();
}
};
}
public void lock() {
QNode qnode = myNode.get();
QNode pred = tail.getAndSet(qnode);
if (pred != null) {
qnode.locked = true;
pred.next = qnode;
// wait until predecessor gives up the lock
while (qnode.locked) {}
}
}
public void unlock() {
QNode qnode = myNode.get();
if (qnode.next == null) {
if (tail.compareAndSet(qnode, null))
return;
// wait until predecessor fills in its next field
while (qnode.next == null) {}
}
qnode.next.locked = false;
qnode.next = null;
}
class QNode {
boolean locked = false;
QNode next = null;
}
}
```
= Problem 10
== (a)
The speedups are listed below:
/ TASLock: 0.1830
/ BackoffLock: 0.2012
/ ReentrantLock: 0.1581
/ CLHLock: 0.8923
/ MCSLock: 0.9041
Yes it does make sense given the complexity of the paths. The implemented locks seem to do much better, since they are able to almost instantly acquire the lock. I was a little surprised by the fact that the more complex locks, such as the CLHLock and the MCSLock went so much faster than their competition, despite having to create and edit objects constantly.
== (b)
#bimg("img/m1.png")
/ Green: TASLock
/ Purple: BackoffLock
/ Blue: ReentrantLock
/ Red: CLHLock
/ Black: MCSLock
It looks like the more complex algorithms start worse, which is to be expected. However they quickly outscale and outperform the simpler algorithms. The TASLock and BackoffLock are the worst performing, which is to be expected since they are the simplest. The ReentrantLock is a little better, but still not as good as the CLHLock and MCSLock. The CLHLock and MCSLock are the best performing, which is to be expected since they are the most complex.
== (c)
#bimg("img/m2.png")
Yes, the correlation matches somewhat with the previous part. However, it is important to notice that there was an incredible amount of variety in the results, and the standard standard deviation deviated by orders of magnitude. I averaged the value over multiple runs to get the best possible average value.
However, with this take into mind, we can see that my tuning of BackoffLock was perhaps a little too good, as it saw an almost out of character placement in the graph. Everything else seemed to follow a reasonable trend through, with the more complex locks having generally lower standard deviation.
|
|
https://github.com/typst/packages | https://raw.githubusercontent.com/typst/packages/main/packages/preview/typearea/0.1.0/typearea.typ | typst | Apache License 2.0 | #let typearea(div: 9, bcor: 0mm, two-sided: true, ..rest, body) = {
let width = 100% - bcor
let height = 100%
set page(
..rest,
margin: if two-sided {
(
"top": height / div,
"bottom": height / div * 2,
"inside": width / div + bcor,
"outside": width / div * 2,
)
// Auto currently defaults to left, as there is no way to check the text language
} else if rest.named().at("binding", default: auto) != right {
(
"top": height / div,
"bottom": height / div * 2,
"left": width / div * 1.5 + bcor,
"right": width / div * 1.5,
)
} else {
(
"top": height / div,
"bottom": height / div * 2,
"left": width / div * 1.5,
"right": width / div * 1.5 + bcor,
)
}
)
body
}
|
https://github.com/arinbasu/typst_docs | https://raw.githubusercontent.com/arinbasu/typst_docs/main/paper.typ | typst | = Human digital twins
== Abstract
|
|
https://github.com/julius2718/entempura | https://raw.githubusercontent.com/julius2718/entempura/main/0.0.1/main.typ | typst | MIT License |
#let sans(it) = {
set text(font: ("Source Sans 3", "Arial"), size: 11pt,)
it
}
#let gtheading(it) = {
set par(first-line-indent: 0em)
text(sans[*#it* #h(1em)])
}
#let today = {
datetime.today().display("[day] [month repr:long] [year]")
}
#let signature(title: "", date: "", author: "") = {
align(center, text(1.4em)[
*#title*
#v(0.3em)
])
align(center, text(1em)[
#date
#v(0.3em)
#author
])
v(0.6em)
}
#let endoc(doc, textfont: "Times New Roman", mathfont: "STIX Two Math") = {
set text(font: (textfont, "Noto Serif JP", "Hiragino Mincho ProN", "BIZ UDPMincho"), size: 11pt,)
show math.equation: set text(font: mathfont)
show raw: set text(font: ("Cascadia Code", "Courier New"), size: 1.1em)
set heading(numbering: "1.")
set math.equation(numbering: "(1.1)")
set page(margin: 1in, numbering: "1")
set par(first-line-indent: 2em, justify: true, leading: 1em)
set block(spacing: 1em)
show heading: it => {
// v(0.6em)
it
par(text(size: 0.3em, ""))
}
let gtheading(it) = {
text(sans[#h(-1em) *#it* #h(1em)])
}
[#doc]
}
#let ensdoc(doc, textfont: "Times New Roman", mathfont: "STIX Two Math") = {
set text(font: (textfont, "Noto Serif JP", "Hiragino Mincho ProN", "BIZ UDPMincho"), size: 10.5pt,)
show math.equation: set text(font: mathfont)
show raw: set text(font: ("Cascadia Code", "Courier New"), size: 1.1em)
set heading(numbering: none)
set math.equation(numbering: "(1.1)")
set page(margin: 1in, numbering: "1")
set par(first-line-indent: 2em, justify: true, leading: 0.8em)
set block(spacing: 0.8em)
show heading: it => {
// v(0.6em)
it
par(text(size: 0.3em, ""))
}
let gtheading(it) = {
text(sans[#h(-1em) *#it* #h(1em)])
}
[#doc]
}
#let aparticle(doc) = {
set text(font: ("Noto Serif", "Source Serif 4", "Times New Roman"), size: 10.5pt,)
show math.equation: set text(font: "STIX Two Math")
show raw: set text(font: ("Cascadia Code", "Courier New"), size: 1.1em)
set page(margin: (top: 1in, rest: 1in), numbering: "1")
set par(first-line-indent: 2em, justify: true, leading: 0.8em)
set block(spacing: 0.8em)
show heading.where(level: 1): it => {
// v(0.6em)
set align(center)
set text(12pt, weight: "bold")
block(smallcaps(it.body))
par(text(size: 0.5em, ""))
}
show heading.where(level: 2): it => {
// v(0.6em)
set align(left)
set text(11pt, weight: "bold")
block(it.body)
par(text(size: 0.5em, ""))
}
show heading.where(level: 3): it => {
// v(0.6em)
set align(left)
set text(10.5pt, weight: "bold")
block(emph(it.body))
par(text(size: 0.5em, ""))
}
let gtheading(it) = {
text(sans[#h(-1em) *#it* #h(1em)])
}
[#doc]
}
|
https://github.com/goshakowska/Typstdiff | https://raw.githubusercontent.com/goshakowska/Typstdiff/main/tests/test_working_types/quoted/quoted_inserted.typ | typst | #quote[I know that I know nothing.]
#quote[Only the dead have seen the end of war.]
#quote[Ignorance, the root and stem of every evil.] |
|
https://github.com/RiccardoTonioloDev/Bachelor-Thesis | https://raw.githubusercontent.com/RiccardoTonioloDev/Bachelor-Thesis/main/structure.typ | typst | Other | // Frontmatter
#include "./preface/firstpage.typ"
#include "./preface/dedication.typ"
#include "./preface/summary.typ"
#include "./preface/table-of-contents.typ"
// Mainmatter
#counter(page).update(1)
#show link: set text(fill: blue.darken(70%), weight: "medium")
#include "./chapters/introduction.typ"
#include "./chapters/pydnet.typ"
#include "./chapters/xinet.typ"
#include "./chapters/attention.typ"
#include "./chapters/pyxinet.typ"
#include "./chapters/results.typ"
#include "./chapters/conclusioni.typ"
// // Glossario
#include "./appendix/glossary.typ"
// Bibliography
#include "./appendix/bibliography/bibliography.typ"
#include "./preface/acknowledgements.typ"
|
https://github.com/tiankaima/typst-notes | https://raw.githubusercontent.com/tiankaima/typst-notes/master/b298e5-sobolev/main.typ | typst | #align(
center,
text(20pt, font: "New Computer Modern Math")[
*Lecture Notes: Sobolev inequalities*
],
)
#align(
center,
text(12pt)[
<NAME>
],
)
#align(
center,
text(12pt)[
#datetime.today().display("[year]-[month]-[day]")
],
)
#set math.equation(numbering: "(1)")
// = Abstract
// In this lecture, we will discuss the following problem:
// - Let $u in W^(1,p)(RR^n)$ be a nonnegative function, and $p > 1$. Show that there exists a constant $C(n, p)$ such that the following inequality holds:$ (integral_ (RR^n) abs(u)^(p^*) dif x)^(1/p^*) <= C(n, p) (integral_ (RR^n) abs(nabla u)^p dif x)^(1/p) $(where $p^*$ is the Sobolev conjugate of $p$. (i.e. $1/p - 1/p^* = 1/n$))
// - Discuss Sobolev inequality
// - Discuss Gagliardo-Nirenberg inequality
// - Discuss Talenti inequality
// #pagebreak()
#text(fill: blue)[
*Text colored in blue were added after class.*
]
= Preknowledge
== Weak derivative
#text(fill: red)[
*From now on, we'll refer to weak derivative as just derivative, and classical derivative as classical derivative.*
]
Weak derivative is introduced to generalize the concept of derivative to functions that are not differentiable in the classical sense.
Using _integration by parts theorem_, we can extend the definition of derivative to a larger class of functions (e.g. $u in L^1(Omega)$).
#rect(fill: yellow.lighten(80%))[
*Definition:*
$u in L^1_"loc" (Omega)$ is said to have a weak derivative $v in L^1_"loc" (Omega)$ if:
$
integral_Omega u(x) phi^prime (x) dif x eq.triple - integral_Omega v(
x
) phi(x) dif x space.quad forall phi in CC_c^infinity (Omega)
$
In this case, we say $v$ is the weak derivative of $u$ and write $v = u^prime$.
*Generalization:*
In $n$ dimensons, and multi-index $alpha = (alpha_1, alpha_2, ..., alpha_n)$, if we have:
$
integral_Omega u(x) D^alpha phi(x) dif x eq.triple (-1)^(abs(alpha)) integral_Omega v(
x
) phi(x) dif x space.quad forall phi in CC_c^infinity (Omega)
$
here $ D^alpha u = (diff^abs(alpha) u) / (diff x_1^(alpha_1) diff x_2^(alpha_2) ... diff x_n^(alpha_n)) $
We say $v$ is the weak derivative of $u$ and write $v = D^alpha u$.
]
*Properties:*
Weak derivatives are well-defined in the sense that:
- if $u$ has a weak derivative $v$, then $v$ is unique.
$ cases(v_1 = D^alpha u, v_2 = D^alpha u) => v_1 eq.triple v_2 space.quad a.e. $
- If $u in C^k(Omega)$, then $D^alpha u = D^alpha u$ (classical derivative)
#pagebreak()
== Holder continuity
#rect(fill: yellow.lighten(80%))[
*Definition:*
$u$ is said to be Holder continuous with exponent $gamma$ if there exists a constant $C$ such that:
$ abs(u(x) - u(y)) <= C abs(x-y)^gamma space.quad forall x,y in Omega $
We write $u in C^(0, gamma)(Omega)$
]
Norm on Holder space is defined as:
$
norm(u)_(C^(0, gamma)(Omega)) = norm(u)_(C^(0) (Omega)) + [u]_gamma = sup u(
x
) + sup_(x != y) abs(u(x) - u(y)) / abs(x - y)^gamma
$
*Properties:*
- A function on an interval satisfying the condition with $gamma > 1$ is constant.
*Proof:*
$
f: I -> Y \
f in C^(0, gamma)(I) => f in C^(0)(I)
$
If $f$ isn't constant, say $x != y, f(x) != f(y)$, then let:
$
x_i(n) &= x + i / n (y - x) space.quad i = 0, 1, ..., n, forall n in NN \
d_Y (f(y), f(x)) &<= sum_(i = 1)^n d_Y (f(x_i(n)), f(x_(i-1)(n))) \
&<= C sum_(i = 1)^n abs(x_i(n) - x_(i-1)(n))^gamma = C sum_(i = 1)^n abs(y - x)^gamma / n^gamma
$
So we have:
$ d_Y (f(y), f(x))<= C abs(y - x)^gamma n^(1-gamma) space.quad forall n in NN $
Since $d_n -> 0 (n -> infinity), 1 - gamma < 0$, we have that, for $epsilon = d_Y (f(y), f(x)) > 0, exists n_0$ that $d_(n_0) < epsilon$, which is a contradiction.
So $forall x, y in I, f(x) = f(y)$, which means $f$ is constant.
- If $gamma = 1$, then the condition is Lipschitz continuity.
- The condition implies uniform continuity.
#pagebreak()
= Note
== Introduction
=== Previous inequalities
- Triangle inequality: $ abs(x + y) <= abs(x) + abs(y) $
- Cauchy inequality: $ abs(x y) <= abs(x) abs(y) $
- Young inequality: $ x y <= x^p/p + y^q/q $ where $1/p + 1/q = 1$, $x, y >= 0$
- Holder inequality: $ integral_ (RR^n) abs(f g) dif x <= (integral_ (RR^n) abs(f)^p dif x)^(1/p) (integral_ (RR^n) abs(g)^q dif x)^(1/q) $ where $1/p + 1/q = 1$
- Jesen inequality: $ f(integral_ (RR^n) g) <= integral_ (RR^n) f(g) dif x $ where $f$ is a convex function
#pagebreak()
=== Background
To introduce the background of Sobolev inequality, we start by discussisng the following problem:
$ Delta u + f = 0 $
Here $f$ might not be continous, might just be $L^2$ function.
To solve this, we first find the minimizer of the following functional:
$ J(u) = 1 / 2 integral_(Omega) abs(nabla u)^2 dif x - integral_(Omega) f u dif x $
($u in CC^(infinity)_c (RR_n)$, meaning that $abs(u != 0) < infinity$)
We take:
$
J(u + epsilon phi) = 1 / 2 integral_(Omega) abs(nabla u + epsilon nabla phi)^2 dif x - integral_(Omega) f (
u + epsilon phi
) dif x
$($u, phi in CC^(infinity)_c (RR_n)$)
When u is the minimizer, we have:
$ dif / (dif epsilon) J(u + epsilon phi) = 0 "at" epsilon = 0 $
$ dif / (dif epsilon) J(u + epsilon phi) = integral_(Omega) nabla u nabla phi dif x - integral_(Omega) f phi dif x $
Consider $ integral nabla u nabla phi + integral Delta u phi = integral_(diff Omega) nabla u dot phi eq.triple 0 $
we have:
$
integral_(Omega) Delta u phi dif x + integral_(Omega) f phi dif x & eq.triple 0, forall phi in CC_0^infinity (RR^n) \
=> Delta u + f &= 0
$
Thus leading to the weak solution of the PDE.
#rect(fill: aqua.lighten(80%))[
#text(fill: red)[
*Question:* When $f in L^2$, when does $integral u dot f$ make sense?
]
#text(fill: blue)[
*When $u in L^2$, $f in L^2$*, using Holder, we have $ integral u dot f <= (integral abs(u)^2)^(1/2) (integral abs(f)^2)^(1/2) < infinity $
]
]
#pagebreak()
=== Sobolev space
Sobolev space is introduced:
$
W^(1,2)(Omega) &= {u in L^2(Omega) | nabla u in L^2(Omega)} \
norm(u)_(W^(1,2)(Omega)) &= (integral abs(u)^2 + integral abs(nabla u)^2)^(1 / 2)
$
_Trivial_: $W^(1,2)(Omega)$ defines normed space.
#text(fill: blue)[
We can also define $W^(1,p)(Omega)$ for $p > 2$ likewise:
$
W^(1,p)(Omega) = {u in L^p (Omega) | nabla u in L^p(Omega)}\
norm(u)_(W^(1,p)(Omega)) = (integral abs(u)^p + integral abs(nabla u)^p)^(1 / p)
$
More generally, we can define $W^(k,p)(Omega)$ for $k in NN$:
$
W^(k,p)(Omega) = {u in L^p (Omega) | D^alpha u in L^p (Omega), forall 0 <= abs(alpha) <= k}\
norm(u)_(W^(k,p)(Omega)) = (sum_(0 <= abs(alpha) <= k) integral abs(D^alpha u)^p)^(1 / p) \
norm(u)_(W^(k, infinity)) = "esssup"_(x in Omega) sum_(0 <= abs(alpha) <= k) abs(D^alpha u(x))
$
_Note:_ Sobolev space is a Hilbert space, and it's a Banach space for $p > 1$.
]
#pagebreak()
== Sobolev inequality
#rect(fill: yellow.lighten(80%))[
If Sobolev inequality holds, we have:
$ norm(f)_(L^(p^*)(Omega)) <= C norm(f)_(W^(1,p)(Omega)) $
That leads to the following statement:
$ f in W^(1,p)(Omega) => f in L^(p^*)(Omega) $
Notice $p^* > p$, thus Sobolev inequality is a statement about the integrability of the function.
]
*Discussions:*
- If and when does Sobolev inequality hold?
- What's the best constant $C(n,p)$?
- What's the extremal function of the inequality?
- Existence
- Expression
Previous works show that:
$ u(x) = 1 / ((a + b dot abs(x)^(p / (p-1)))^((n-p) / p)) $
$
C(n,p) = 1 / (sqrt(pi)n^(1 / p))((p-1) / (n-p))^(1-1 / p) (
(Gamma(1 + n/2)Gamma(n)) / (Gamma(1 + n - n/p)Gamma(n/p))
)^(1 / n)
$
#rect(fill: aqua.lighten(80%))[
#text(fill: red)[
*Question:* For $1/p^* = 1/p - 1/n$ to hold, we must have $p<n$, what happens when $p=n$?
]
#text(fill: blue)[
*Theorem: $forall q >= n$, $ norm(u)_(L^q (RR^n)) <= (omega_(n-1))^(1/q - 1/n) ((q + 1 - q/n)/n)^(1/q - 1/n +1) norm(u)_(W^(1,n)(RR^n)) $where $omega_(n-1) = abs(SS^(n-1))$, volume of the unit sphere in $RR^n$.*
]
]
Approximation of the constant:
$ ((q + 1 - q / n) / n)^(1 / q - 1 / n +1) tilde.op q^((n-1) / n) (1 / n)^((n-1) / n) $
#text(fill: red.lighten(50%))[
_The $1/n$ here is actually $1/n - 1/n^2$, I'm not sure if I've missed something or just doesn't matter._
]
Given by Trudinger in 1967, we have:
$ norm(u)_(L^q (RR^n)) <= C q^((n-1) / n) norm(u)_(W^(1,n)(RR^n)) $
We can show that:
$
integral e^u &= sum_(j=0)^infinity 1 / (j!) integral u^j = sum_(j=0)^infinity 1 / (j!) norm(u)_(L^j)^j\
&<= sum_(j=0)^infinity 1 / (j!) (C dot j^((n-1) / n) norm(u)_(L^n))^j\
&= C sum_(j=0)^infinity j^(j dot (n-1) / n) / (j!) norm(u)_(L^n)^j\
$
Using Strling's formula ($n! tilde.op n^n/e^n sqrt(2 pi n)$) , we have:
$ j^(j dot (n-1) / n) / (j!) tilde.op 1 / (e^(-j + j / n) n^(j / n + 1 / 2) (j / n)!) <= C^prime 1 / ((j / n)!) $
Rewrite the sum, we have:
$
sum_(j=0)^infinity j^(j dot (n-1) / n) / (j!) norm(u)_(L^n)^j <=C^' sum_(j=0)^infinity norm(u)_(L^n)^j / ((j / n)!)
= C' e^(norm(u)_(L^n)^n)
$
Thus we have:
$ integral e^u <= C e^(norm(u)_(L^n)^n) $
#rect(fill: yellow.lighten(80%))[
This is called Orlicz-Sobolev inequality. Orlicz space is defined as:
$ L^Phi (Omega) := {u in L^1(Omega) | integral Phi(abs(u)/lambda) dif x <= 1} $
In 1971, Moser proved that:
$
sup_(norm(nabla u)_(L^n) <= 1) integral e^(alpha_n u^(n / (n-1))) <= infinity
$ ($u in CC_0^infinity (Omega), alpha_n = n omega_(n-1)^(1/(n-1)), omega_(n-1) := abs(SS^(n-1))$)
]
Before we continue, let's prove the following inequalities:
#pagebreak()
=== Gagliardo-Nirenberg-Sobolev inequality
#rect(fill: yellow.lighten(80%))[
_Goal:_ Say $1<= p < n$, there exisits $C(n,p)$ such that:
$
norm(u)_(L^(p^*)(RR^n)) <= C norm(D u)_(L^p (RR^n)) space.quad forall u in W^(1,p)(RR^n)
$
]
*Proof:*
_Step 1:_ $p = 1, p^* = n/(n-1)$
$
abs(u(x)) &= abs(integral_(- infinity)^(x_i) diff _i u(x_1, ..., x_i, ..., x_n) dif x_i)
&<= integral_(- infinity)^infinity abs(nabla u) dif x_i
$
$
abs(u(x))^(n / (n-1)) &<= (product_(i=1)^n integral_(- infinity)^x_i abs(nabla u) dif x_i)^(1 / (n-1)) \
integral_(- infinity)^infinity abs(u(x))^(n / (n-1)) dif x_1
&<= integral_(- infinity)^infinity (
product_(i=1)^n integral_(- infinity)^x_i abs(nabla u) dif x_i
)^(1 / (n-1)) dif x_1 \
&= (integral_(-infinity)^infinity abs(nabla u) dif x_1)^(1 / (n-1)) integral_(- infinity)^infinity (
product_(i=2)^n integral_(- infinity)^infinity abs(nabla u) dif y_i
)^(1 / (n-1)) dif x_1 \
&<= (integral_(-infinity)^infinity abs(nabla u) dif x_1)^(1 / (n-1)) product_(i=2)^n (
integral_(- infinity)^infinity integral_(- infinity)^infinity abs(nabla u) dif x_1 dif y_i
)^(1 / (n-1)) \
$
Repeating this process, it's trivial to show that:
$
integral_(RR^k) abs(u)^(n / (n-1)) dif x_1 dif x_2 ... dif x_k \
<= product_(i=1)^k (integral_(RR^k) abs(nabla u) dif x_1 dif x_2 ... dif x_k)^(1 / (n - 1))
product_(i=k+1)^n (integral_(RR^(k+1)) abs(nabla u) dif x_1 dif x_2 ... dif x_k dif y_i)^(1 / (n - 1))
$
Subsitute $k = n$, we have:
$
integral_(RR^n) abs(u)^(n / (n-1)) dif x &<= (integral_(RR^n) abs(nabla u) dif x)^(n / (n - 1)) \
(integral_(RR^n) abs(u)^(n / (n-1)) dif x)^((n - 1) / n) &<= integral_(RR^n) abs(nabla u) dif x \
$
_Step 2:_ $1 < p < n$
Subsitute $u$ with $u^gamma$, we have:
$
(integral_(RR^n) abs(u)^((gamma n) / (n-1)) dif x)^((n - 1) / n) &<= integral_(RR^n) abs(nabla abs(u)^gamma) dif x \
&= gamma integral abs(u)^(gamma - 1) abs(nabla u) dif x \
&<= gamma (integral abs(u)^(((gamma -1) p) / (p - 1)) dif x)^((p-1) / p) (integral abs(nabla u)^p dif x)^(1 / p)
$
Last inequality uses Holder inequality.
Letting $(gamma n)/(n-1) = ((gamma -1)p)/(p - 1)$, we have $ gamma = (p(n - 1))/(n - p)$, thus:
$(gamma n)/(n-1) = ((gamma -1)p)/(p - 1) = p^*$, leading to the final result.
#pagebreak()
#text(fill: blue)[
=== Morrey inequality
]
#rect(fill: yellow.lighten(80%))[
_Goal:_ Assuming $n < p <= infinity$. Then there exists a constant $C= C(n,p)$ (depending only on $n$ and $p$, not with $u$), for every $u in C^1(RR^n) sect L^p (RR^n)$, we have:
$ norm(u)_(C^(0,gamma)(RR^n)) <= C norm(u)_(W^(1,p)(RR^n)) space.quad gamma = 1 - n / p $
Thus: if $u in W^(1,p) (RR^n)$, then $u$ is Holder continuous with exponent $gamma = 1 - n/p$.(after a possible redefinition on a set of measure zero)
]
*Proof:*
$forall x in RR^n, rho > 0, z in diff B_1, 0 < t < rho$,
$ abs(u(x+t z) - u(x)) = abs(integral_0^t dif / (dif s) u(x + s z) dif s) <= integral_0^t abs(nabla u(x + s z)) dif s $
$
integral_(diff B(0, 1)) abs(u(x+t z) - u(x)) dif z &<= integral_0^t integral_(diff B(
0, 1
)) abs(nabla u(x + s z)) dif z dif s \
&= integral_(B(0, 1)) abs(nabla u(y)) / (abs(x - y)^(n - 1)) dif y
$
$
integral_(B (x, rho)) abs(u(y) - u(x)) dif y &= integral_0^rho dif s integral_(diff B(x, s)) abs(u(y) - u(x)) dif y \
&= integral_0^rho s^(n-1) dif s integral_(diff B(0, 1)) abs(u(x + s z) - u(x)) dif s \
&<= integral_0^rho s^(n-1) dif s integral_(B(x, rho)) abs(nabla u(y)) / (abs(x - y)^(n-1)) dif s \
&= rho^n / n integral_(B(x, rho)) abs(nabla u(y)) / (abs(x - y)^(n-1)) dif s
$
Setting $rho = 1$, we have:
$
abs(u(x)) &<= 1 / abs(B(0,1)) (integral_(B(0,1)) abs(u(x) - u(y)) + abs(u(y)) dif y) \
&<= 1 / n integral_(B(0,1)) abs(nabla u(y)) / (abs(x - y)^(n-1)) dif y + 1 / abs(B(0,1)) integral_(B(
0,1
)) abs(u(y)) dif y \
&<= C_1 norm(nabla u)_(L^p) + C_2 norm(u)_(L^p (B(0,1))) \
&<= C norm(u)_(W^(1,p) (RR^n))
$
Equation holds for every $x$, thus we have:
$
sup_(x in RR^n) abs(u(x)) <= C norm(u)_(W^(1,p) (RR^n))
$
Estamating $abs(u(x) - u(y))$, take $rho = abs(x - y), O = B(x, rho) sect B(y, rho)$,
$
abs(u(x) - u(y)) <= 1 / abs(O) integral_O abs(u(x) - u(z)) dif z &+ 1 / abs(O) integral_O abs(u(y) - u(z)) dif z \
1 / abs(O) integral_O abs(u(x) - u(z)) dif z
&<= C 1 / abs(B(x, rho)) integral_(B(x, rho)) abs(u(x) - u(z)) dif z \
&<= C 1 / abs(B(x, rho)) rho^n / n integral_(B(x, rho)) abs(nabla u(z)) / (abs(x - z)^n) dif z \
&<= C rho^(1- n / p) norm(nabla u)_(L^p (B(x, rho)))
$
Given all that,
$
norm(u)_(C^(0, gamma) (RR^n)) <= C norm(u)_(W^(1,p) (RR^n)) space.quad gamma = 1-n / p
$
#pagebreak()
= Homework
== Question 1.1
#rect(fill: yellow.lighten(80%))[
Why the power on the left hand side has to be such $p^*$
#text(fill: red)[
Hint: try replace $u(x)$ by $u(lambda x)$, after change of variables, you will see that in order to guarantee that Sobolev inequality are independent of the scaling of $u$, the power on the left hand side has to be $p^*$
]
]
== Answer
#text(fill: blue)[
Subsitute $u$ with $u(lambda x)$, we have
$
"LHS" = (integral_(RR^n) abs(u(x))^(p^*) lambda^n dif x)^(1 / p^*)
= lambda^(n / p^*) (integral_(RR^n) abs(u(x))^(p^*) dif x)^(1 / p^*)
$
$
"RHS" = C(n, p) (integral_(RR^n) abs(nabla u(x))^p lambda^(p-n) dif x)^(1 / p)
= lambda^(n / p - 1) C(n, p) (integral_(RR^n) abs(nabla u(x))^p dif x)^(1 / p)
$
Since $C(n,p) tilde.not u(x) \/ u(lambda x)$, we need $lambda^(n/p^*) eq.triple lambda^(n/p - 1) => n/p^* = n/p - 1$, which gives $1/p^* = 1/p - 1/n$.
]
== Question 1.2
#rect(fill: yellow.lighten(80%))[
It has been shown during class that the extremal functions of Sobolev inequality should satisfy the following semilinear PDE:
$ - "div"(abs(nabla u)^(p-2) nabla u) = C abs(u)^(p^*-2) u $
On the other hand, applying the moving plane method, we can show the positive solution of the above equation has to be radially decreasing. Combinging the two results, please try to reduce the above equation to a second order ODE and solve it:
]
== Answer
#text(fill: blue)[
// Using spherical coordinates, we have
$
// nabla u &= ((diff u)/(diff r), 1/r nabla_theta u) \
-"LHS" &= nabla(abs(nabla u)^(p-2)nabla u) = (p-2)abs(nabla u)^(p-2)laplace u + abs(nabla u)^(p-2) laplace u = (p-1)abs(nabla u)^(p-2)laplace u \
&= - C abs(u)^(p^*-2) u
$
Deducing to ODE: (and taking $p = 2, u > 0$)
$
(diff^2 u) / (diff x^2) = -C u^(2^* - 1)
$
General solution for this ODE is:
$
u =
$
]
#text(fill: red)[
#strike[
Haven't come up with a solution yet, will update later
]
]
#pagebreak()
== Question 2
#rect(fill: yellow.lighten(80%))[
Assuming the Sobolev inequalties:
$ norm(u)_(L^q (Omega)) <= C q^((n-1) / n) norm(u)_(L^n(Omega)) $
holds for any $q >= 1$, try to prove the following exponential type inequality (will need to use Stirling formula):
$
integral_Omega e^u dif x <= C e^(mu norm(u)_(L^n)^n) space.quad C, mu "are some constants"
$
]
== Answer
#text(fill: blue)[
_This is already proven in previous sections, copying the proof here for completeness:_
We can show that:
$
integral e^u &= sum_(j=0)^infinity 1 / (j!) integral u^j = sum_(j=0)^infinity 1 / (j!) norm(u)_(L^j)^j\
&<= sum_(j=0)^infinity 1 / (j!) (C dot j^((n-1) / n) norm(u)_(L^n))^j\
&= C sum_(j=0)^infinity j^(j dot (n-1) / n) / (j!) norm(u)_(L^n)^j\
$
Using Strling's formula ($n! tilde.op n^n/e^n sqrt(2 pi n)$) , we have:
$ j^(j dot (n-1) / n) / (j!) tilde.op 1 / (e^(-j + j / n) n^(j / n + 1 / 2) (j / n)!) <= C^prime 1 / ((j / n)!) $
Rewrite the sum, we have:
$
sum_(j=0)^infinity j^(j dot (n-1) / n) / (j!) norm(u)_(L^n)^j <=C^' sum_(j=0)^infinity norm(u)_(L^n)^j / ((j / n)!)
= C' e^(norm(u)_(L^n)^n)
$
Thus we have:
$ integral e^u <= C e^(norm(u)_(L^n)^n) $
Notice you could just take $C^prime = C e^mu$ for a cleaner formula.
]
|
|
https://github.com/polarkac/MTG-Stories | https://raw.githubusercontent.com/polarkac/MTG-Stories/master/stories/054%20-%20Lost%20Caverns%20of%20Ixalan/005_Episode%205.typ | typst | #import "@local/mtgstory:0.2.0": conf
#show: doc => conf(
"Episode 5",
set_name: "Lost Caverns of Ixalan",
story_date: datetime(day: 20, month: 10, year: 2023),
author: "<NAME>",
doc
)
== Quint
What would it be like to touch the sun?
Quint stood on a hill overlooking the valley that housed the Thousand Moons garrison and the nearby town, gently fanning his face with his ears. The land curved upward in the distance rather than remaining flat, while below, aqueducts wound their way between clusters of pyramids and smaller buildings, the water they carried pooling in stone reservoirs. He watched the Sun Empire warriors don special harnesses, supervised by members of the Thousand Moons. Huatli cooed to her bat, while Wayta and her mount already made lazy circles in the air.
Steward Akal seemed to sense Quint's envy. "This is a coming-of-age ceremony for our people," she said. "Our new cousins are already of age, and have their own traditions, but we are glad to share this with them."
"I'm honored to observe," Quint said. "Besides, someone has to stay with Pantlaza."
Hearing his name, the raptor peered over to Quint. Once he realized Quint had no food, he went back to his nap.
The bat riders launched into the air, gradually shrinking to black dots as they approached the glowing pink tail stretching from the broken shell around the sun. The cosmium reef, they called it, a dangerous place filled with metal shards and fragments of the crystals used in their armor, weapons, and other devices. Huatli and the others would try to claim a piece of that cosmium for themselves.
Steward Akal interrupted Quint's reverie. "You are from another plane? Not the surface?"
"I am," Quint replied. "Arcavios. I attended a university there, Strixhaven."
"A place of learning?" <NAME> asked.
"Yes," Quint said. "I studied archaeology and archaeomancy, among other things. I want to find lost stories and share them. Preserve them for future generations."
<NAME> gave a quiet hum of acknowledgment. "You are like our didacts. They explore and learn and teach. Stories are the memory of the plane, and to forget is to succumb to darkness. Even during the Night War, our stories were a guiding light."
Quint pondered how to ask about the Night War, or the metal around the sun, or any of a million other questions. There was so much to learn here, he hardly knew where to begin.
He returned his attention to the bats. "Is this meant to be secret, or could I share this with my colleagues?"
"Not secret," <NAME> said. "But it would be best to leave explanations to those of us who understand the nuances. I would be happy to summon one of our didacts to assist you, perhaps in exchange for stories and knowledge from your own home?"
"An exchange would be excellent." A didact might also be able to help with his Coin Empire research. Depending on how things progressed, he could bring Saheeli here soon, or some of his colleagues from Arcavios …
A breeze cooled Quint's skin and set the grassy fields rippling. Quint pulled his goggles down over his eyes to get a better view. Dinosaurs and other animals roamed wild, rodents the size of dogs and long-necked ruminants with soft-looking fur. So peaceful.
The distant figures darting among the reef began to return. Huatli was in front, Inti behind her, while Caparocti and Wayta trailed after them with the other soldiers.
#figure(image("005_Episode 5/01.png", width: 100%), caption: [Art by: <NAME>], supplement: none, numbering: none)
Huatli landed first, dismounting and handing her bat to an attendant. She all but bounced toward <NAME>, waving a chunk of pink crystal.
"I found a fragment!" Huatli exclaimed. "Is it large enough for a necklace, do you think?"
St<NAME> nodded. "You might also be able to adorn a weapon with it. A sword, perhaps?"
A shadow crossed Huatli's face. "Perhaps," she said, her enthusiasm dampened. She scratched Pantlaza's feathered head, earning a happy trill in response.
Inti and Caparocti glided to a halt, but Wayta flew past. A piercing whistle sent the Oltec riders chasing her, including Chara, and Quint wondered if she might be having trouble with her mount. Within a span of breaths, however, she turned back and landed, but didn't climb down. The brow over her visible eye was furrowed, her mouth set in a grim line that mirrored the tension in her shoulders.
"There," Wayta said with deliberate calm, pointing toward the far end of the garrison across the valley. "The vampires have summoned a cloud of their cursed fog, bigger than usual."
Quint didn't know what that meant, but the rest of the Sun Empire faction tensed.
"You're sure?" Caparocti asked.
Wayta nodded. Inti cursed under his breath. Another sharp whistle with a different cadence announced Chara's return. She, like Wayta, stayed mounted.
"The Thousandth Moon approaches," Chara said. "She has two prisoners with her."
<NAME> leaned on her staff. "We must question them."
The warriors muttered among themselves as Wayta dismounted and stood at Quint's side. The grim form of Anim Pakal stalked toward them, weapon ready, gnome automaton at her side. In front of her, a vampire and a man trudged along wearily. The vampire stumbled and the man caught her, helping her regain her footing.
"We found these two running from the garrison," Anim told her sister.
"We weren't escaping," the man said defensively. "We were trying to find help." Quint peered at him and realized his ears were pointed. An elf, he guessed, the first he'd seen on Ixalan.
"I'm Amalia, and this is Kellan," the vampire said. "The other vampires, they … They killed our human servants and the queen's emissary, then escaped. They're looking for Aclazotz."
"The Betrayer?" Anim asked, recoiling with shock. "He's been imprisoned for ages."
"Do you know where he is?" Amalia asked.
<NAME> paled and clutched her staff more tightly. "No, but some among the Oltec still worship him, and they may have passed this knowledge down." She looked toward the sun, closing her eyes. "If the vampires find these allies, they may at last be powerful enough to release the bat god from his prison. No one in the Core will be safe from his unending blood thirst."
Just what we need, Quint thought. A violent god trying to kill everyone for fun.
#v(0.35em)
#line(length: 100%, stroke: rgb(90%, 90%, 90%))
#v(0.35em)
== Malcolm
A host of fungal creatures closed in as the Mycotyrant peered down at Malcolm and Breeches from its webbed perch in the cavern, the green glow in its eyes inscrutable.
"Enough slow talk," it said, still using Xavier as a puppet. "We will assimilate you, and we will ascend to the surface and its sun."
Malcolm mentally plotted how quickly he could fly away with Breeches, and whether it would matter when the only exit was guarded.
There must be another way out. He stretched his senses to feel the air currents and found a tunnel behind the Mycotyrant. A faint breeze from that direction carried the scent and sensation of a large body of water. Here? Underground?
"#emph[BIG BOOM] ?" Breeches asked, his whisper awkwardly loud.
Malcolm glanced upward, then around, his gaze falling on a mushroom-encrusted dinosaur. He remembered the fight in the cavern and smiled.
"Let me try something first," Malcolm said. "Plug your ears."
Breeches obeyed. Taking a deep breath, Malcolm began to sing.
The Mycotyrant's eyes closed and everyone around Malcolm froze, stunned. He continued to sing, grabbing Breeches and tugging him toward the exit that led to water. They passed more unmoving figures, and even the toadstool trees seemed quiescent. He wondered if his proximity to the Mycotyrant made his magic affect the entire colony, as the creature called its horde of puppets.
He found the tunnel, glad for his shoulder light since the glowing fungus had darkened. Breeches kept his hands over his ears as he followed. Malcolm wasn't sure how long his magic would work, but he sang until they were deep into the rock, hoping it would carry and keep the monsters in his sway until he and Breeches were safe.
A hideous, many-mouthed scream echoed behind them. He really needed to stop hoping.
"Run," Malcolm said, then took off in a dead sprint.
Breeches ran, his ambling gait keeping pace with Malcolm's. Green light erupted around them, fungal growths twisting and reaching for them as they passed. He didn't dare look back for fear it would slow him down enough to be caught.
The feel and scent of water strengthened. A light loomed ahead, more blue than green, with faint tingles of magic interspersed. River Herald, but also something else. Something older and more powerful.
Without warning, the tunnel ended in a short cliff. Below him, an ocean stretched so far he couldn't sense the end of it, while closer to his position, a gilded city crowded the banks and descended into the depths. Merfolk swam with purpose or lingered on the shore, some guards pointing up at him and Breeches while others continued their business unaware of the impending incursion.
"Incoming!" Malcolm shouted, imbuing magic in his voice to make it carry. He grabbed Breeches and dove out over the water, angling toward the city.
The Mycotyrant's forces erupted from the tunnel. Malcolm finally risked a glance backward. It seemed every infected resident of Downtown dogged his heels, tumbling onto the beach or splashing awkwardly into the water. Their numbers were dwarfed by half-decayed dinosaurs and cat-people, and those creepy walking mushrooms, some wielding weapons while others gathered magic to themselves. Worse, holes in the roof of the cavern spouted flying creatures, dinosaurs and giant bats so encrusted with fungus they struggled to stay aloft.
The chaos of battle destroyed any serenity the place had enjoyed. Merfolk drew weapons, activated the enchantments in their armor, pulled out jade totems and summoned huge elemental creatures to repel the fungal invasion. Walking bonfires flung gouts of flame, while hulking waterspouts knocked foes from the air, sending them tumbling end over end to their dooms.
At the top of a pyramid like those in the Sun Empire, a door opened into a space that promised impossible skies, if Malcolm and Breeches could only reach it …
#figure(image("005_Episode 5/02.png", width: 100%), caption: [Art by: <NAME>], supplement: none, numbering: none)
A merfolk warrior loomed in front of Malcolm, brandishing a jade-tipped staff. He skidded to a stop.
"Who are you?" she asked.
"<NAME>, at your service," Malcolm said, giving a polite bow. "This is Breeches, my associate."
Breeches tipped his hat.
"Pirates," the merfolk said, mouth twisting with distaste. "I am Nicanzil. What evil have you brought to our shores?"
"Calls itself the Mycotyrant," Malcolm said. "Turns people into mushrooms."
Nicanzil pondered this, her fins shifting behind her. "This must be the danger the Sun Empire mentioned. Our people beyond the golden door must be warned that it is closer than they knew."
If warning them meant escaping through that door, Malcolm was ready to oblige. "Come on," he told Breeches. "Let's go sound the alarm."
Breeches bared his teeth in assent as he drew his three swords—one for each hand and one with his tail—and together they traversed the battlefield, dodging combatants as Malcolm aimed unerringly for the promise of open skies.
#v(0.35em)
#line(length: 100%, stroke: rgb(90%, 90%, 90%))
#v(0.35em)
== Vito
#emph[Come to me …]
The voice of Aclazotz called to Vito more clearly than ever. He led the remaining vampires of his expedition through the broad paths of the garrison, shrouded in impenetrable fog. Despite Bartolomé thwarting his intended sacrifice, he'd finally had his revenge on that thorn of the Black Rose. His body thrummed with the holy sacrament he'd consumed, and anticipation of more to come.
Voices called out in the mist, laced with confusion and bravado and, more than anything, fear. Clavileño and the other soldiers found and silenced some, gorging themselves in an ecstasy of blood. The words of Venerable Tarrian thrummed in Vito's mind like the heartbeats of the people he stalked.
#emph[Blessed are the weak, for they shall feed the strong.]
#emph[Blessed are the peaceful, for they shall yield without struggle.]
#emph[Blessed are the merciless, for they shall require no mercy.]
A red glow appeared in the fog, walking toward Vito. He lowered his lance to attack.
"<NAME>," the mysterious figure whispered. "I have been sent to guide you to Aclazotz."
Could this be a trick? Surely no one would dare.
"I am ready," Vito said, raising the lance like a standard.
They left the garrison and trampled across carefully tended fields, past isolated homesteads whose denizens huddled together in fear, finally descending into the depths of a fetid swamp. The vampires' skin was as pale as the moss hanging like the beards of old men from the gnarled branches around them. Boots squelched in ankle-high mud that stank of rotten eggs and decay, and any animal sounds and songs that might once have given life to the land fell silent as if under a spell. More people joined them, carrying or wearing crystals that glowed a dim red, until a veritable army traveled unseen within the fog that wound its way between trees and turned the sun into a feeble ghost. Some of them dragged glassy-eyed prisoners through the muck, presumably imminent sacrifices for Aclazotz.
"Who are you?" Vito asked.
"We are the servants of Aclazotz," the figure replied. "We have worshipped our master since he was imprisoned, waiting for the day of his salvation."
Vito faltered at the mention of imprisonment. He had assumed Aclazotz merely bided his time, part of a greater plan—not that he had been trapped here, in this nightless place. The father of vampires, caged.
Then, a rush of pride overcame that flicker of doubt. He was the instrument of his god, a savior. He would realize the vision of Venerable Tarrian, exceeding the disappointment that was Saint Elenda. A new, pure era would begin in Torrezon, with him leading the faithful.
Vito didn't know how long he trudged through the swamp. Once, he might have been surprised his enemies hadn't found him, riding their bats through the skies. Surely the fog would be visible from a distance. But Aclazotz worked in mysterious ways, and Vito trusted his god to ensure the success of his chosen saint.
Before he could ask how much longer until they reached their destination, the fog cleared, revealing the mouth of a cave. Nothing about it marked the location as special; no signs or symbols were carved into the exposed stone, no temple steps invited entry, no gold or silver adorned the entrance or the tunnel beyond. It might have been any cave in any place in all the plane, and Vito knew its anonymity was part of its power.
Vito had lost the magical candles that lit his way through the caverns of the underground. The red lights of the servants of Aclazotz guided him, first through a narrow corridor that might have been naturally formed, then into a side tunnel that had clearly been clawed open according to some intelligent design. The stench of rot pervaded the space, as if this were a midden or charnel house rather than the shrine of a deity.
He would see Aclazotz properly worshipped in temples befitting His Majesty, and his foes would suffer for their impertinence.
The tunnel ended in a strange door, ringed in symbols and engravings he didn't recognize, a gaping hole at its center. The figure who led him retrieved something from their robes, a chunk of pink crystal that glowed with an inner light.
"This is the key," they said, handing it to Vito. "Place it inside and be marked as worthy … or be destroyed."
Vito did not doubt his worth. He took the crystal and placed it in the cavity.
A ring of stones clamped down on his arm, rendering him unable to move. The pink glow of the crystal brightened, and with it came a searing pain, as if his fist had been plunged into the sun. Vito clenched his teeth until his fangs pierced his lip, blood trickling down his chin. The sensation shifted from fire to ice as the flush of vitality from his recent feedings drained from him, pouring into the cavity until he trembled, more enervated than he'd felt since his last Blood Fast. His knees threatened to buckle, but he forced them to straighten and bear his weight. He would not fail Aclazotz, now or ever.
The pain and pressure vanished, and Vito pulled his arm free. Crimson light spread to the glyphs around the door, and it rolled sideways with a deep rumbling sound.
"You may enter the sanctum," said his guide.
A proper temple facade awaited, its columns rising to four times Vito's height. A stream of bats erupted from the entrance, as if to mark the occasion of his arrival. He waited for them to pass, then continued his procession, Clavileño and the others behind him like an honor guard.
#figure(image("005_Episode 5/03.png", width: 100%), caption: [Art by: <NAME>], supplement: none, numbering: none)
A small antechamber opened into a vast amphitheater with tiered rows of seating. Carved glyphs in the walls bathed the assembled crowds in blood-red light, and a thousand pale faces turned to watch Vito enter. He lifted the lance of Tarrian higher, descending to meet his fate, to reach the figure at the center of the room.
"Come to me," said the voice, as familiar to Vito now as his own name.
Aclazotz.
The bat god crouched on the floor, shrouded by his wings and wrapped in loops of thick golden chains inlaid with hundreds of pink crystals. His body was the brown of old, dried blood, wings gilded and tattered where they brushed the stone beneath him. A collar of skulls hung from his neck, and a black and gold crown adorned his head, proclaiming his godhood to any who gazed upon him.
A single red eye pierced Vito with the strength of its regard, and he fell to his knees in worship.
"My master," Vito murmured, voice thick with emotion. "I have come."
Aclazotz drew a deep, rustling breath. "My slumber was disturbed by the invaders on the surface," he said. "And so, I have called to you all, my children of the night. The time of my ascension is at hand."
"We shall rise," the assembled cosmium eaters intoned.
"The end of the Fifth Age is upon us," Aclazotz continued. "Chimil's light will be extinguished, and my darkness will be absolute. You, my chosen, will serve at my side, glorying in the salvation of life everlasting. Bring forth the sacrifices."
A wail emerged from a cave to Vito's left. The people who'd been dragged through the swamp were penned inside, huddled together like lambs at market. Vito rose and approached them, gesturing for his soldiers to attend him.
"Do not fear death," Vito said soothingly. "Your blood will give rise to the power, glory, and eternal kingdom of Aclazotz, now and forever."
One by one, the prisoners were delivered unto the bat god, who feasted on their essences as Vito respectfully averted his gaze. The bodies were dragged away and thrown into a pit, faint streaks of gore marking their passing. With every death, the baleful eye of the god brightened, a beacon in the dim room.
And then it was done. Aclazotz huddled in on himself, then with a piercing scream, he rose and strained against his bonds. The pink crystals in the chains flickered madly, then dulled to the same red as the god's eye. A concussive burst of magic struck everyone in the room as the gold links snapped in a dozen places. With a mighty shrug, Aclazotz sent the chains scattering across the floor. He lifted himself to his full height and stretched his wings, and Vito fell to his knees again in awe.
"Come to me," Aclazotz intoned. "Receive my blessing."
Vito reached the god first, prostrating himself. "I am worthy," he said.
"Then my eternal covenant shall be yours."
Aclazotz bent down until his breath misted Vito's face, smelling of blood and some sickly-sweet flower in the throes of decay. The fangs of the god lengthened and sharpened. In a flash, he sank them deep into Vito's neck and torso. Vito screamed. Pain spread across his chest, then into his limbs, a fire beyond anything blood had ever ignited in his veins. Muscles spasmed and bones cracked and reformed, black spots dancing across his vision as unconsciousness threatened to claim him. The fangs of Aclazotz released him, and he dimly sensed that the others around him were being blessed as well, one after another.
#figure(image("005_Episode 5/04.png", width: 100%), caption: [Art by: <NAME>], supplement: none, numbering: none)
The magic receded, and Vito winced at the profusion of sounds and scents that suddenly demanded his attention. The dim red lights of the crystals blazed like torches even as all other colors were leached out of the room. His once pristine robes now hung in tatters from his larger frame, torn by his transformation and the ministrations of Aclazotz. He swiveled new ears toward Clavileño, who had finished his own transformation and turned beady eyes to Vito.
Where once vampires had knelt, new creatures stood, their forms echoing their master's. They retained their armor and weapons, but their hands now ended in vicious claws. Vito flexed his wings, longer fangs protruding from his enlarged mouth.
"Come," Aclazotz said. He launched himself into the air, exiting the room through a broad hole in the ceiling.
Vito followed without hesitation, his comrades beneath him. They passed through a dark tunnel made bright by the echoes of their collective shrieks, until a pinprick of distant light grew to reveal the world beyond. They emerged from the caves, a legion prepared to do battle for the god who had gifted them with his power. The first flash of the sun's brilliant glow stung his eyes, but they soon adjusted, and the land spread out before him in shades of stark black, bone white, and misty gray. Air currents swirled around them, carrying myriad scents both familiar and unknown—the rich decay of the swamp, the musk of animals, moisture-laden clouds, and, just ahead, bats and humans mingling together. Up they flew, higher and further, until they reached the long trail of pink-tainted metal that arced away from the sun.
Aclazotz hovered, his eye once again flaring as he turned his gaze to the light. "Chimil," he whispered, addressing the Core's shining sun. "As I was consigned to my prison, so shall you be. I will consume your precious Oltec as I did their ancestors, as I consumed your feeble god-spawn to close the veil between death and life. I will end the Fifth Age at last, and my children will bring the Sixth Age to the plane."
His largest wings stretched wide, then began to close, slowly, straining as if he were moving an impossibly heavy weight.
The metal around the sun shifted, pieces rotating and locking together like a broken container being reassembled. Which was precisely what it was: Aclazotz wielded his power to reform a shattered sphere that would soon imprison Chimil.
#figure(image("005_Episode 5/05.png", width: 100%), caption: [Art by: <NAME>], supplement: none, numbering: none)
The shadow of that prison fell across the land, enshrouding it in a darkness deeper than any night in Torrezon. Vito's altered eyes thrilled at the change, the richness of the landscape's textures both heard and seen.
With a harsh gasp, Aclazotz closed his wings and fell to the ground, impacting with a sound like thunder. Vito landed beside him as the god struggled to stand, glaring at the remaining shafts of light escaping the sun's metal walls.
"More," Aclazotz said. "I require more sacrifices. You will bring them to me."
"Thy will be done," Vito said. He shrieked his command to the assembled Legion, and their response echoed through the mountains until it shook the foundations of the plane.
#v(0.35em)
#line(length: 100%, stroke: rgb(90%, 90%, 90%))
#v(0.35em)
== Wayta
The sun darkened, and Wayta fought the urge to panic, cold sweat pooling in the small of her back.
She rode her bat toward the clouds of fog that covered the Thousand Moon garrison and the swamps and mountains beyond. They'd lost time searching the villages and outlying areas for the escaped vampires, finding Oltec huddled in their homes and signs of violence in the streets. Their destination had been uncertain until a swarm of creatures shot out of a cave, led by a bat as large as three or four people standing end to end: Aclazotz.
At first, Wayta assumed the monsters with him were other bats, but her spyglass told another story: they were vampires, corrupted somehow, turned into abominations that resembled their foul god, yet still wearing their armor and carrying their weapons. The Legion made straight for the cosmium reef, halting at its edge. Before the first among the Oltec could reach them, the light had begun to disappear as if a shade were being drawn over a lantern.
The war against the Phyrexians had been like this. Day suddenly turning to night as the enemy blotted out the sun. The screams of her allies, her friends, turned to sobs, pleas, prayers—then cut off. The wave of memories crested, and she rode it like a ship in a storm, trying not to capsize.
Huatli glided up to her, riding her own bat, her shard of the Threefold Sun illuminating her face as it had in the darkest caverns.
"Breathe, little warrior," Huatli shouted. "Victory will be ours this day."
Was it day anymore? It didn't matter. The sentiment held, even if she'd heard it uttered by so many who wouldn't live to see another hour.
"Chimil's prison is being remade," The flight leader shouted from her mount. "We must stop the betrayer Aclazotz, or Chimil will be sealed inside again."
Wayta didn't want another war. Not with a so-called god, not with the sun-forsaken vampires, not with anyone. But she would fight this battle, the one before her, the one forced on her. And she would win.
#figure(image("005_Episode 5/06.png", width: 100%), caption: [Art by: <NAME>], supplement: none, numbering: none)
The crystals on the flight leader's headpiece gleamed like a pink constellation, as did similar gems on the other bat riders. Below, across the land, in garrisons and villages, cities and huts, people called light to themselves. Single flames and bonfires, flickering glows and steady beacons. Wayta didn't know these Oltec well yet, but she felt a kinship with them, with this refusal to bow to the weight of shadow.
"Still think we should leave Torrezon alone?" Caparocti shouted at Huatli.
"This isn't Torrezon," she replied, dark eyes cold. "Even so, these monsters will never see their homeland again once we reach them."
"There's the warrior-poet we all know and love," Inti joked. "Give us a poem before we ride into battle."
Caparocti rolled his eyes, but Wayta listened as best she could, not wanting the words to be lost to the wind that whistled past.
Huatli's gaze grew distant, then shifted to meet Wayta's. When she spoke, it was loud, defiant.
#emph[We do not fear the little mist]
#emph[We have weathered hurricanes]
#emph[We do not fear the falling night]
#emph[We rise with the Threefold Sun]
"For the Threefold Sun!" Caparocti shouted. The Sun Empire troops echoed his words, and the Oltec bat riders trilled a ferocious whistle that sent shivers up Wayta's neck.
A terrible shriek echoed from the Legion. Some of the bats careened wildly or bucked, nearly dropping their riders. Wayta's mount shrilled back and dove, her stomach leaping into her throat as she struggled to regain control.
The vampire abominations flew toward the Oltec force.
Huge bat wings kept these strange creatures aloft. Beady eyes stared from gaunt, pale faces with flattened noses and huge ears. Some still wore their helms, some their plate armor, while others must have once been Oltec given their torn ponchos and khipu. They shrieked again as the vanguard of riders reached them, spears and bright pink magic colliding with swords and sickly waves of red.
One of the Dusk Legion charged Wayta, brandishing his sword. She drew her own, thumbing the magic stone that extended it into a spear. She waited, biding her time, focus narrowing to take in speed and distance. The vampire-bat was nearly in striking distance when she urged her mount into a shallow dive, striking upward. Her blade tore a deep gash in her enemy's wing, and with a scream, he spun toward the earth.
Nearby, Huatli chased a pair of cosmium eater bats, while one of the Thousand Moons fought a creature with a tall, familiar-looking lance. Wayta moved to flank, but another vampire bore down on her. She banked her bat to avoid his sword, then barely missed another attack from her blind side. With an annoyed grunt, she pulled her mount upward to survey the battle from above. The swirls of the cosmium reef floated around her, drifts of crystals small as drops of rain. It would have been beautiful, if the blood of her allies and enemies wasn't joining the cloud.
So it would always be, she thought darkly. Blood in the sky, blood on stone, blood in the rivers and in the foam on the sea.
A golden gleam caught her eye, glinting in the slivered light of the sun. The door at the base of the mountain, the one through which they'd passed to enter this new land. Someone flew out of it—another vampire-bat? No, a siren? One she was almost sure she recognized.
A rush of greenish blackness poured through the portal behind him. Fungal creatures, like the ones she and the other Sun Empire warriors had fought in the deserted city by the underground river. Hundreds of them, perhaps thousands.
All of them preparing to swarm the garrison.
"It always rains when it's wet," Wayta muttered.
#v(0.35em)
#line(length: 100%, stroke: rgb(90%, 90%, 90%))
#v(0.35em)
== Malcolm
The open air was not the balm Malcolm had hoped for, despite its relative sweetness after so long slogging through stagnant caves. His enemies dogged his heels, tossing spore bombs and casting ropes of fungus to tie people to the ground. Worse, as intriguing as this new land might be, sloping upward to encircle the sky, there appeared to be another battle already in progress.
Malcolm put more distance between himself and the teeming mass of sentient mushrooms, trying to keep an eye on Breeches. The goblin's three blades spun and cut like a reaper mowing a field, but he would be overcome if reinforcements didn't manifest soon.
The closer Malcolm flew to the strange, shadowed sun at the center of this place, the more the air currents shifted—no, slowed, as if some magic force made them drowsy. His feathers fluffed slightly, the weight of his limbs easing barely enough to notice, and yet he did. It was incredible. It drew him like a moth to flame, or a fish to a hook.
In the floating reef of pink-infused metal, people riding bats warred with—people-bats? Wearing Dusk Legion armor? He blinked at that in confusion. Some of the riders were Sun Empire, if their armor and the lights they carried were any indication, but how had they found this place? And why?
One of them caught his eye: a familiar face from High and Dry, or rather, a recognizable weapon and eyepatch. She had moved away from the center of the fight and now stared in his direction. Malcolm raced toward her, and she did the same, each fighting the occasional bat-winged vampire until the two of them met in the middle.
"Wayta," Malcolm said, relieved. "What is going on here?"
"Let me explain," Wayta said, then paused. "No, there is too much. Let me sum up. The vampires have transformed into bat monsters and their god imprisoned the sun. You?"
"A giant mushroom is infecting people and turning them into its puppets so it can take over the plane."
Wayta snorted. "It'll have to get in line. Do you think it could infect a god?"
"Let's not find out," Malcolm said, his feathers rippling with unease.
"Follow me," Wayta said. "We must warn the steward, if she doesn't already know."
She led him up—no, down—to a cluster of humans dressed in unfamiliar garb, as well as a vampire and two strangers. Off-worlders, like Vraska and Jace had been, if he had to guess. And those cursed Phyrexians.
One of those off-worlders jogged over immediately, a loxodon with goggles on his head. "Wayta!" he exclaimed breathlessly. "Everything alright up there? What's happening? Who's your friend?"
Wayta grinned. "Quint, this is <NAME>. Everything's a mess, and there's a horde of those walking mushrooms pouring through the golden door."
Quint seemed to think this through. "How many is a horde?"
"How many mushrooms make a forest?" Malcolm replied.
"Mushrooms?" the pointy-eared young man asked. "Are they poisonous?"
Malcolm laughed grimly. "Much worse. They're ambitious."
The vampire woman sighed. "Saints protect us from ambition."
Someone else approached, a woman wearing an elaborate headdress and leaning on a staff. "This is quite a time of strife," she said. "I am <NAME>, and you seem to have brought us more trouble."
"Not on purpose," Malcolm said. "I came to solve the mystery of what happened to my people, and unfortunately, I found the answer. It said you call it the Mycotyrant."
<NAME> pursed her wrinkled lips. "My sister was mobilizing the Thousand Moons to mount a defense, but between this and Aclazotz, our forces will be spread thin." She gestured at a soldier standing nearby, who ran over and saluted. "Send another urgent summons through the towers to any available gardeners."
Malcolm sighed in relief. This wasn't over, but he and Breeches weren't alone in their fight anymore.
Breeches. Oh no.
"Wayta," Malcolm said. "Can you help me give someone a ride?"
#v(0.35em)
#line(length: 100%, stroke: rgb(90%, 90%, 90%))
#v(0.35em)
== Vito
This battle would be immortalized in the new scriptures of Aclazotz for the rest of time.
Vito dodged a wave of magic from an Oltec bat rider, then speared her through the midsection with his lance. Only her harness kept her from falling to her death as she slumped over the saddle. The scent of her blood sweetened the air, and Vito shrieked in triumph.
Around him, streams of glittering pink dust darkened as the sacrifices converted the power of the reef, bending it to the will of Aclazotz. Soon, the god would finish what he had begun, and the treacherous light of this inner world would be extinguished.
His soldiers pursued their foes relentlessly, looping through the sky, striking human and bat alike. Clavileño had initially formed ranks, but soon the fight transformed into a frenzied melee. Sword fought spear, teeth and claws tore through spells, and the transformed creatures alternately feasted and carried humans back to Aclazotz. The one Vito had struck succumbed to that very fate as a hulking vampire in a torn poncho shredded her harness and pulled her from her mount. Her scream faded into the darkness, and Vito moved on to his next opponent.
Clavileño soared past, trading sword strikes with a burly Sun Empire warrior wielding a staff with a blade on each end. The weapon knocked Clavileño's helmet off, and the vampire barely ducked a second slash that would have opened his throat. Instead, it sliced his ear in half. Either Clavileño felt no pain, or it didn't bother him, because he renewed his attack with greater ferocity.
Before Vito could intervene, another warrior descended on him, armed with a shield and a broad, long sword. This one he remembered from the introductions among the River Heralds: Inti, seneschal of the sun. How appropriate for him to meet his end here, as Aclazotz snuffed out this sun's light.
"For the power and glory of Aclazotz!" Vito growled, launching himself at the interloper.
Inti's shield knocked Vito's lance away, his sword slashing down toward Vito's neck. Vito glided back, the blow whistling harmlessly through empty air. He stabbed again with his lance, and again his attack was deflected. They circled each other in the air, sun and shadow, fire and smoke. The seneschal moved his shield to expose the light on his belt, temporarily blinding Vito, who retreated with a hiss.
"Don't like the power of the Threefold Sun, bloodsucker?" Inti taunted, pushing his temporary advantage by unleashing a flurry of cuts. He didn't notice Clavileño gliding silently toward him.
Vito bared his newly elongated fangs. "My god granted me something greater than yours, weakling."
"What is that?" Inti asked.
"The power of flight." Vito drove his lance down in a single, powerful thrust—straight into the skull of Inti's mount. The bat shuddered and went limp, then began to fall, with Inti still harnessed to its saddle.
The warrior fought to unhook the latches that tethered him to the mount, and in doing so left himself vulnerable. Clavileño circled behind him and gripped Inti's head in one massive claw.
"Glory to Aclazotz," Vito said. Clavileño snapped Inti's neck and both human and bat bodies fell into darkness.
"Inti!" A scream from nearby. The warrior-poet on her own mount, diving toward her fallen comrade.
Vito followed her. She, too, would make an excellent sacrifice.
|
|
https://github.com/JosephBoom02/Appunti | https://raw.githubusercontent.com/JosephBoom02/Appunti/main/Anno_3_Semestre_1/Controlli%20Automatici/equation_numbering.typ | typst | #let equation_numbering(doc) = {
set heading(numbering: "1.")
show heading.where(level: 1): h => {
h
// reset equation counter for each chapter
counter(math.equation).update(0)
}
set math.equation(numbering: eqCounter => {
locate(eqLoc => {
// numbering of the equation: change this to fit your style
let eqNumbering = numbering("a", eqCounter)
// numbering of the heading
let chapterCounter = counter(heading).at(eqLoc)
let chapterNumbering = numbering(
// use custom function to join array into string
(..nums) => nums
.pos()
.map(str)
.join("."),
..chapterCounter
)
// change this to fit your style
[(#chapterNumbering - #eqNumbering)]
})
})
doc
} |
|
https://github.com/typst/packages | https://raw.githubusercontent.com/typst/packages/main/packages/preview/tgm-hit-thesis/0.1.0/CHANGELOG.md | markdown | Apache License 2.0 | # [v0.1.0](https://github.com/TGM-HIT/typst-diploma-thesis/releases/tags/v0.1.0)
Initial Release
|
https://github.com/LucaCiucci/tesi-triennale | https://raw.githubusercontent.com/LucaCiucci/tesi-triennale/main/README.md | markdown | Creative Commons Zero v1.0 Universal | # tesi-triennale (Physics bachelor thesis)
Slides for my bachelor thesis in Physics.
This repository contains the code for the slides of my bachelor thesis in Physics.
[](https://github.com/LucaCiucci/tesi-triennale/releases/tag/final)
See the [final release page](https://github.com/LucaCiucci/tesi-triennale/releases/tag/final) to download the PDF.
## Compilation
```sh
typst compile main.typ main.pdf --input final=true
typst compile main.typ main-with-script.pdf
typst compile main.typ out-svg/{n}.svg --input final=true
```
Dev:
```sh
typst watch main.typ
start .\main.pdf
``` |
https://github.com/donabe8898/typst-slide | https://raw.githubusercontent.com/donabe8898/typst-slide/main/opc/並行prog/03/Go3.typ | typst | MIT License | #show link: set text(blue)
#set text(font: "Noto Sans CJK JP",size:13pt)
#show heading: set text(font: "Noto Sans CJK JP")
#show raw: set text(font: "0xProto Nerd Font")
#show raw.where(block: true): block.with(
fill: luma(245),
inset: 10pt,
radius: 10pt
)
#align(center)[
```go
// 今回はコメント書かなくてOKです
package main
import "fmt"
func main() {
// 3大都市の連想配列
mp := map[string]bool{
"Tokyo": true,
"Osaka": true,
"Nagoya": false,
}
fmt.Printf("%v\n", mp) // 一旦表示してみる
mp["Fukuoka"] = false // 福岡を追加
fmt.Printf("%v\n", mp)
delete(mp, "Nagoya") // 名古屋は3大都市引退したほうが良いと思う(爆)
fmt.Printf("%v\n", mp)
fmt.Println("長さ=", len(mp)) // mapの長さを確認
_, ok := mp["Osaka"] // 大阪が存在するかどうか
if ok {
fmt.Println("ok")
} else {
fmt.Println("Not found")
}
for k, v := range mp {
fmt.Printf("%s=%t\n", k, v)
}
}
```
]
|
https://github.com/Bzero/typstwriter | https://raw.githubusercontent.com/Bzero/typstwriter/master/README.md | markdown | Other | # 
An integrated editor for the [typst](https://github.com/typst/typst) typesetting system.

## Features
* Integrated Document Viewer
* Integrated File System viewer
* Syntax highlighting
## Configuration
Typstwriter can be configured using a configuration file, see [example_config.ini](docs/example_config.ini) for an example config file. After modifying the config file, typstwriter needs to be restarted.
Typstwriter looks for configuration files in the following locations:
* /etc/typstwriter/typstwriter.ini
* /usr/local/etc/typstwriter/typstwriter.ini
* ~/.config/typstwriter/typstwriter.ini
* ~/.typstwriter.ini
* ./typstwriter.ini
Files further down in the list will take precedence.
## Installation
### Using pip:
Typstwriter is available on [PyPI](https://pypi.org/project/typstwriter/). To install using `pip` simply use:
```
pip install typstwriter
```
### From source
Get the source code by cloning the desired branch or download a release from th github repository and install from the toplevel directory:
```
git clone https://github.com/Bzero/typstwriter
pip install ./typstwriter/
```
### Install typst
Independently of the installation method chosen, the `typst` CLI has to be available to compile typst documents. Consult the [typst repository](https://github.com/typst/typst#installation) for details on installing `typst`. Alternatively, the path of a `typst` executable can be specified in the [configuration file](#Configuration).
## Development
Clone the repository
```
git clone https://github.com/Bzero/typstwriter/
```
Install typstwriter in edit mode
```
pip install -e ./typstwriter/
```
Run the tests with pytest to make sure that everything is working correctly. Inside the source directory, run:
```
pytest
```
Activate pre-commit hooks to automatically check changes before committing. Inside the source directory, run:
```
pre-commit install
```
## Contributing
Contributions are always welcome!
* Open an [Issue](https://github.com/Bzero/typstwriter/issues) to report bugs or request features.
* Use the [discussion](https://github.com/Bzero/typstwriter/discussions) to give feedback.
* Send a [Pull request](https://github.com/Bzero/typstwriter/pulls) to propose new features.
## License
Typstwriter is licensed under the [MIT license](LICENSE).
|
https://github.com/r8vnhill/apunte-bibliotecas-de-software | https://raw.githubusercontent.com/r8vnhill/apunte-bibliotecas-de-software/main/Unit2/Lateinit.typ | typst |
== Null-safety: Inicialización tardía
A veces no es posible inicializar una variable en el momento de su declaración sin comprometer la
limpieza del código o la eficiencia. Para estos casos, Kotlin ofrece dos herramientas útiles:
`lateinit` y `Delegates.notNull()`.
=== Uso de `lateinit`
- *Propósito*: La palabra clave `lateinit` permite declarar variables no nulas que serán
inicializadas posteriormente. Es especialmente útil cuando una variable depende de inyección de
dependencias o configuración inicialización que no está disponible en el momento de la creación
del objeto.
- *Aplicabilidad*: Solo se puede usar con variables de tipos que son inherentemente no nullables
y que no son primitivos (como `Int`, `Float`, etc.).
- *Verificación*: Puedes verificar si una variable `lateinit` ha sido inicializada mediante
`::variable.isInitialized` antes de acceder a ella para evitar errores en tiempo de ejecución.
```kotlin
class Player {
lateinit var name: String // Declaración de una variable que se inicializará más tarde
fun initializePlayer(name: String) {
this.name = name
}
}
```
=== Uso de `Delegates.notNull()`
- *Propósito*: `Delegates.notNull()` es una forma de crear una propiedad que debe ser inicializada
eventualmente, y se utiliza cuando las variables son de tipo primitivo o cuando `lateinit` no es
aplicable.
- *Comportamiento*: Acceder a una propiedad delegada por `notNull()` antes de su inicialización
lanzará una `IllegalStateException`, indicando que la propiedad no ha sido inicializada.
```kotlin
class Player {
var lifePoints: Int by Delegates.notNull() // Delegación para asegurar la inicialización antes de uso
}
```
#line(length: 100%)
*Ejercicio: Implementación de un Perfil de Usuario*
Desarrolla una clase `UserProfile` que administre la información de un usuario, utilizando inicialización tardía para ciertos campos.
Descripción de la Clase:
- *Campos con Inicialización Tardía:* La clase `UserProfile` debe incluir dos campos que se inicializarán tardíamente:
- `username`: Una cadena de texto (`String`) que representa el nombre de usuario.
- `age`: Un entero (`Int`) que representa la edad del usuario.
- *Requisitos Funcionales:*
- *Función de Visualización de Información:* Define una función `displayUserInfo()` dentro de la clase que:
- Imprima los datos del usuario (nombre de usuario y edad) si ambos campos han sido inicializados.
- Muestre un mensaje de error si el nombre de usuario no se ha inicializado
#line(length: 100%) |
|
https://github.com/exAClior/touying-simpl-hkustgz | https://raw.githubusercontent.com/exAClior/touying-simpl-hkustgz/master/README.md | markdown | MIT License | # Touying Slide Theme for HKUST GZ
Inspired by [Touying Slide Theme for Beihang University](https://github.com/Coekjan/touying-buaa)
## Use as Typst Template Package
Use `typst init @preview/touying-simpl-hkustgz` to create a new project with this theme.
```console
$ typst init @preview/touying-simpl-hkustgz
Successfully created new project from @preview/touying-simpl-hkustgz:<latest>
To start writing, run:
> cd touying-simpl-hkustgz
> typst watch main.typ
```
## Examples
See [examples](examples) and [Github Pages](https://exaclior.github.io/touying-simpl-hkustgz) for more details.
You can compile the examples by yourself.
```console
$ typst compile ./examples/main.typ --root .
```
And the PDF file `./examples/main.pdf` will be generated.
## License
Licensed under the [MIT License](LICENSE). |
https://github.com/typst/packages | https://raw.githubusercontent.com/typst/packages/main/packages/preview/cades/0.2.0/lib.typ | typst | Apache License 2.0 | #import "@preview/jogs:0.2.0": compile-js, call-js-function
#let qrcode-src = read("./qrcode.js")
#let qrcode-bytecode = compile-js(qrcode-src)
#let qr-code(
content,
width: auto,
height: auto,
color: black,
background: white,
) = {
let result = call-js-function(qrcode-bytecode, "qrcode", content, color.to-hex(), background.to-hex())
return image.decode(result, width: width, height: height)
} |
https://github.com/crd2333/Astro_typst_notebook | https://raw.githubusercontent.com/crd2333/Astro_typst_notebook/main/src/docs/here/test/第三方包.typ | typst | ---
order: 3
---
#import "/src/components/TypstTemplate/lib.typ": *
#show: project.with(
title: "This is a title",
// show_toc: false,
lang: "zh",
)
Pay attention to the order.
This file has order: $3$.
从第三方包中(or自己编写)预先挑选了一些比较实用的工具,比如:
= Fletcher
Typst 中的 cetz 就像 LaTeX 中的 tikz 一样,提供强大的画图功能,但是个人感觉略繁琐。#link("https://github.com/Jollywatt/typst-fletcher")[Fletcher] 基于 cetz 提供了 diagrams with arrows 的简单画法。
#import fletcher.shapes: diamond
#diagram(
node-stroke: .1em,
node-fill: gradient.radial(blue.lighten(80%), blue, center: (30%, 20%), radius: 80%),
spacing: 4em,
edge((-1,0), "r", "-|>", [open(path)], label-pos: 0, label-side: center),
node((0,0), [reading], radius: 2em),
edge([read()], "-|>"),
node((1,0), [eof], radius: 2em),
edge([close()], "-|>"),
node((2,0), [closed], radius: 2em, extrude: (-2.5, 0)),
edge((0,0), (0,0), [read()], "--|>", bend: 130deg),
edge((0,0), (2,0), [close()], "-|>", bend: -40deg),
)
#align(center, grid(
columns: 3,
gutter: 8pt,
diagram(cell-size: 15mm, $
G edge(f, ->) edge("d", pi, ->>) & im(f) \
G slash ker(f) edge("ur", tilde(f), "hook-->")
$),
diagram(
node-stroke: 1pt,
edge-stroke: 1pt,
node((0,0), [Start], corner-radius: 2pt, extrude: (0, 3)),
edge("-|>"),
node((0,1), align(center)[
Hey, wait,\ this flowchart\ is a trap!
], shape: diamond),
edge("d,r,u,l", "-|>", [Yes], label-pos: 0.1)
),
diagram($
e^- edge("rd", "-<|-") & & & edge("ld", "-|>-") e^+ \
& edge(gamma, "wave") \
e^+ edge("ru", "-|>-") & & & edge("lu", "-<|-") e^- \
$)
))
= syntree & treet
语法树,像这样,可以用字符串解析的方式来写,不过个人更喜欢后一种自己写 `tree` 的方式,通过合理的缩进更加易读。
#let bx(col) = box(fill: col, width: 1em, height: 1em)
#grid(
columns:2,
gutter: 4em,
syntree(
nonterminal: (font: "Linux Biolinum"),
terminal: (fill: red),
child-spacing: 3em, // default 1em
layer-spacing: 2em, // default 2.3em
"[S [NP This] [VP [V is] [^NP a wug]]]"
),
tree("colors",
tree("warm", bx(red), bx(orange)),
tree("cool", bx(blue), bx(teal)))
)
#tab 文件夹型的树,像这样
#tree-list(root: "root")[
- 1
- 1.1
- 1.2
- 1.2.1
- 2
- 3
- 3.1
- 3.1.1
]
= 伪代码(算法)
lovelace包,可以用来写伪代码,body 最好用 typ,比如:
#algo(
caption: [caption for algorithm],
```typ
#no-number
*input:* integers $a$ and $b$
#no-number
*output:* greatest common divisor of $a$ and $b$
<line:loop-start>
*if* $a == b$ *goto* @line:loop-end
*if* $a > b$ *then*
$a <- a - b$ #comment[and a comment]
*else*
$b <- b - a$ #comment[and another comment]
*end*
*goto* @line:loop-start
<line:loop-end>
*return* $a$
```
)
= wrap_content
文字图片包裹,不用自己考虑分栏了。在大多数时候是比较有效的,但有的时候不是很好看,可能还是得自己手动 grid。
#let fig = figure(
rect(fill: teal, radius: 0.5em, width: 8em),
caption: [A figure],
)
#let body = lorem(40)
#wrap-content(
align: bottom + right,
column-gutter: 2em,
fig
)[
#indent #body
]
#wrap-top-bottom(fig, fig, body)
= 真值表
快速制作真值表,只支持 $not and or xor => <=>$。
#truth-tbl($A and B$, $B or A$, $A => B$, $(A => B) <=> A$, $ A xor B$)
#tab 更复杂的用法(自己填data),三个参数分别是样式函数、表头、表内容:
#truth-tbl-empty(
sc: (a) => {if (a) {"T"} else {"F"}},
($a and b$, $a or b$),
(false, [], true, [] , true, false)
)
= todo(checklist)
- [ ] 加入更多layouts,比如前言、附录
- [x] 重构代码,使得可以根据语言切换文档类型
= Pinit
#v(2em)
$ (#pin(1)q_T^* p_T#pin(2))/(p_E#pin(3))#pin(4)p_E^*#pin(5) >= (c + q_T^* p_T^*)(1+r^*)^(2N) $
#pinit-highlight-equation-from((1, 2, 3), 3, height: 3.5em, pos: bottom, fill: rgb(0, 180, 255))[
In math equation
]
#pinit-highlight-equation-from((4, 5), 5, height: 1.5em, pos: top, fill: rgb(150, 90, 170))[
price of Terran goods, on Trantor
]
`print(pin6"hello, world"pin7)`
#pinit-highlight(6, 7)
#pinit-point-from(7)[In raw text]
#v(4em)
这玩意儿的用法略灵活,可以看它的仓库 #link("https://github.com/typst/packages/tree/main/packages/preview/pinit/0.1.4")[pinit]
= mitex
使用 #link("https://github.com/typst/packages/tree/main/packages/preview/mitex/0.2.4")[mitex] 包渲染 LaTeX 数学环境,比如:
通过这个包,可以快速把已经在 Markdown 或 LaTeX 中的公式重复利用起来;同时,利用市面上众多的 LaTeX 公式识别工具,可以减少很多工作。
#mitex(`
\newcommand{\f}[2]{#1f(#2)}
\f\relax{x} = \int_{-\infty}^\infty
\f\hat\xi\,e^{2 \pi i \xi x}
\,d\xi
`)
#mitext(`
\iftypst
#set math.equation(numbering: "(1)", supplement: "equation")
\fi
A \textbf{strong} text, a \emph{emph} text and inline equation $x + y$.
Also block \eqref{eq:pythagoras}.
\begin{equation}
a^2 + b^2 = c^2 \label{eq:pythagoras}
\end{equation}
`)
|
|
https://github.com/SWATEngineering/Docs | https://raw.githubusercontent.com/SWATEngineering/Docs/main/src/2_RTB/VerbaliInterni/VerbaleInterno_231121/content.typ | typst | MIT License | #import "meta.typ": inizio_incontro, fine_incontro, luogo_incontro
#import "functions.typ": glossary, team
#let participants = csv("participants.csv")
= Partecipanti
/ Inizio incontro: #inizio_incontro
/ Fine incontro: #fine_incontro
/ Luogo incontro: #luogo_incontro
#table(
columns: (3fr, 1fr),
[*Nome*], [*Durata presenza*],
..participants.flatten()
)
= Sintesi Elaborazione Incontro
== Frequenza degli aggiornamenti alla Proponente
Il team si impegna a mantenere la Proponente costantemente informata sull'avanzamento durante lo sprint, con un aggiornamento settimanale. Tale aggiornamento avverrà idealmente a metà sprint; tuttavia, in caso di urgenze o necessità particolari, il team contatterà la Proponente per ottenere il loro feedback in momenti aggiuntivi. Inoltre, comprendendo l'interesse dei referenti della Proponente nell'analizzare il codice sviluppato prima della Sprint Retrospective, il team si impegna a contattarli a tal proposito anche poco prima della fine dello sprint.
== Numerosità dei Verificatori
Il team ha valutato l'assegnazione del ruolo di Verificatore, decidendo di limitarlo a un solo membro per sprint successivi al primo. Questa scelta mira a garantire una maggiore enfasi e supporto ai ruoli le cui mansioni sono attualmente più pressanti, come il Programmatore o l'Analista.
Tuttavia, nel caso si decida in futuro di coinvolgere più di un Verificatore, la politica per assegnare loro le issue da revisionare all'interno dell'ITS è la seguente: il Verificatore seleziona autonomamente la issue con la priorità maggiore all'interno della "corsia" Ready to Review sulla Kanban Board in utilizzo su Github e procede con la revisione.
== Pianificare date di inizio e fine per ogni _issue_
Al termine di ogni meeting interno la prassi è quella di creare e assegnare le issue all'interno dell'ITS, consentendo la definizione di parametri come la dimensione (Size) e la priorità (Priority) di ciascuna. In data odierna si è deciso di concordare anche la data di inizio e soprattutto di fine di ogni issue, al fine di pianificare l'avanzamento del lavoro in maniera più dettagliata, considerando scadenze più immediate rispetto alla fine dello sprint corrente. Questa pratica mira a facilitare il monitoraggio delle interconnessioni tra le diverse issue e favorire una collaborazione più efficace tra i membri del team, permettendo una pianificazione più precisa e agevolando la gestione delle dipendenze tra le varie attività.
== Lavorare in team di due persone
Il team si sta orientando verso un approccio collaborativo dove, durante lo sviluppo del codice, due o più Programmatori lavorano insieme, anche se non in un'esclusiva modalità di pair-programming su una singola issue. Questo approccio mira a favorire lo scambio di consigli e la collaborazione diretta, ma non implica necessariamente un impegno costante su una singola attività.
== Data di scadenza del RTB
Il team ha fissato come termine ultimo di consegna del RTB il 18 dicembre 2023, poco dopo il termine dei primi due sprint e mezzo (che ricoprono il periodo che va dal 10 novembre 2023 al 15 dicembre 2023). È stata prevista una finestra aggiuntiva di qualche giorno per valutare approfonditamente il lavoro completato e per condurre le revisioni necessarie.
== Spostamento dei meeting interni
I meeting interni attualmente pianificati per ogni martedì verranno spostati al venerdì (il giorno della settimana in cui si conclude lo sprint corrente) per consentire una pianificazione più accurata degli obiettivi dello sprint successivo e una conseguente assegnazione dei ruoli. Pertanto, il meeting settimanale si terrà il venerdì alle ore 10:30 a metà sprint (in assenza del meeting di Sprint Retrospective tenuto con la Proponente) e alle ore 11:30 alla conclusione dello sprint (subito dopo lo _Sprint Retrospective_).
== Avanzamento del Piano di Progetto
Il Responsabile corrente si incarica della redazione delle prossime sezioni del _Piano di Progetto_, incluse l'Analisi dei Rischi e il Modello di sviluppo. Il team ha ufficialmente concordato sull'adozione di una metodologia agile per la gestione del progetto. Questo perché le pratiche attuate nelle ultime settimane, come sprint, Sprint Planning, Daily Scrum e Sprint Retrospective, riflettono appieno le caratteristiche dinamiche del modello Scrum.
== Avanzamento dell'Analisi dei Requisiti
Il team ha deciso di sottoporre i casi d'uso in forma testuale a una prima verifica esterna durante il meeting di Sprint Retrospective del 24 Novembre. Inoltre, si è pianificato di creare i corrispondenti diagrammi UML tramite draw.io. Durante questa sessione di verifica, il team intende chiarire con la Proponente alcuni dubbi riguardanti l'_Analisi dei requisiti_, in particolare per quanto riguarda il ruolo dei simulatori di sensori (si intende capire se essi rappresentino attori esterni al sistema o siano da considerare componenti interni al sistema stesso). |
https://github.com/yhtq/Notes | https://raw.githubusercontent.com/yhtq/Notes/main/几何学/main.typ | typst | #import "../template.typ": *
// Take a look at the file `template.typ` in the file panel
// to customize this template and discover how it works.
#show: note.with(
title: "几何学",
author: "YHTQ",
date: none,
logo: none,
)
= 前言
- 考核:作业10%,期中30%,期末60%
- 课程主页:www.math.pku.edu.cn/teachers/wangjj/2023fall/index.html
- 邮箱:<EMAIL>
- 答疑:周一5-6,周四5-6,理科一号楼14100
- 几何:研究空间结构和性质的学科
- 空间:集合
- 结构:集合的性质
= 欧氏几何
== 度量空间与欧氏几何
#definition[][
(度量空间)若 $X$ 是集合,$d:X times X -> R$,满足:
+ 非负性:$d(X,Y)>=0$
+ 非退化性:$d(X,Y)=0 <=> X=Y$
+ 对称性:$d(X,Y)=d(Y,X)$
+ 三角不等式:$d(X,Y)<=d(X,Z)+d(Z,Y)$
则称 $(X, d)$ 为一个度量空间, $d$ 为 $X$ 上的一个度量
]
注:
- 这些性质(除了非负性和三角不等式)都是互相独立的
- 显然一个集合上不只有一个度量
- 几何性质往往不依赖于集合本身的性质或者说具体的模型
例:用坐标表示 $n$ 维欧氏空间的点,记作$EE^n = {(x_1,x_2,...,x_n)|x_i in RR,i=1,2,3...,n}$,便可规定度量:$d(X,Y)=sqrt((x_1-y_1)^2+(x_2-y_2)^2+...+(x_n-y_n)^2)$,称为欧氏空间上的典范度量
合理的几何性质应该和具体的坐标系选取没有关系,因此自然产生了等距变换的规定
#definition[等距变换][
若 $X$ 和 $Y$ 是度量空间,$f:X->Y$是双射并且满足:\
$d_Y (f(X),f(Y))=d_X (X,Y)$\
则称 $f$ 是一个等距变换,并称度量空间 $X, Y$ 等距同构。
显然等距同构是等价关系,从而同构保证了不同模型间性质的等价性。\
]
#definition[欧氏几何][
所有与 $n$ 维典范欧氏空间等距同构的度量空间均称为 $n$ 维欧氏几何(本课程中多使用欧式几何)
]
在二/三维欧式平面典范模型中,可以很容易的定义并证明欧几里得几何的诸多概念的公理、定理,再次说明了同构的概念\
- 线段:$overline(P Q)$\
- 有向线段:$arrow(P Q)$\
#example[][
$EE^2$ 中圆盘
$
B_r={(x, y) in EE^2 | x^2+y^2<=r^2}
$
可以证明:
+ $B_r$ (在典范度量下)是一个度量空间
+ 若 $r eq.not s$,则 $B_r$ 与 $B_s$ 不等距同构
]
== 向量代数
通过欧式几何的典范模型,可以将欧氏几何代数化。\
#definition[向量][$EE^3$ 或 $EE^n$ 中有向线段称为向量或矢量,记作 $arrow(P Q)$, 其中 $P$ 称为起点,$Q$ 称为终点。
- 若 $arrow(P Q)$ 与 $arrow(C D)$ 可通过平移重合,则看作相等的向量(向量是有向线段在平移下的等价类)]
#definition[向量空间][空间中向量的全体称为向量空间]
#definition[反向量、零向量][......]
#definition[平行][......]
#definition[向量加法][三角形法则......]
#proposition[][向量加法满足:
+ 交换律
+ 结合律
+ 零向量是加法零元:$bold(a) + 0 = bold(a)$
+ 负向量是加法逆元:$bold(a) + (-bold(a)) = 0$]
换言之,向量关于加法构成交换群,从而可以定义减法
向量有唯一的方向与长度,故可以定义数乘
#definition[][
给定 a 是向量,$lambda$ 是实数,则 $lambda a$ 是向量,称为 $a$ 的数乘,满足:
- 若 $lambda >= 0$,则 $lambda a$ 与 $a$ 同向;若 $lambda <= 0$,则 $lambda a$ 与 $a$ 反向
- $|lambda a| = |lambda| |a|$
显然这样定义的 $lambda a$ 是存在且唯一的
]
#proposition[][数乘满足:
+ $1 dot a = a$, $-1 dot a = -a$
+ (结合律)$(lambda mu) a = lambda (mu a) $
+ (分配律)$lambda (a + b) = lambda a + lambda b$
]
#definition[向量共线/面][
设 $a_i ,space i=1,2,3...,n$ 是向量。若将它们取同一起点后,它们全部落在一条直线/平面上,则称这些向量共线/共面
]
#definition[线性相关][
设 $a_i ,space i=1,2,3...,n$ 是向量。若存在不全为零的实数 $lambda_i ,space i=1,2,3...,n$ 使得:
$
sum_(i=1)^n lambda_i a_i = 0
$
则称这些向量线性相关。反之则称其线性无关。
]
== 仿射标架
#theorem[][
向量 $a, b$ 共线当且仅当存在不全为零的实数 $lambda, mu$,使得 $lambda a + mu b = 0$。\
]
#theorem[][
向量 $a_1, a_2, a_3$ 共面当且仅当存在不全为零的实数 $lambda_i$,使得 $sum_(i=1)^3 lambda_i a_i = 0$。\
]
#corollary[][
$EE^2$ 中任意向量可被两不共线向量线性表出;
$EE^3$ 中任意向量可被三不共面向量线性表出\
]
#definition[(空间)仿射标架][
$EE^3$ 中一个仿射标架指 $(O,e_1,e_2,e_3)$,其中 $O in EE^3$ 是原点,$e_1,e_2,e_3$ 是三个不共面(线性无关)的向量,称为基向量
]
#proposition[][
取定一个仿射标架,对任意 $EE^3$ 中向量 $a$,存在唯一的 $lambda_i$ 使得:
$
a = sum_(i=1)^3 lambda_i e_i
$
此时 $vec(lambda_1,lambda_2,lambda_3)$称为 $a$ 在该仿射标架下的(仿射)坐标
]
#corollary[][
设 $a, b$ 坐标分别为 $autoVec3(a), autoVec3(b)$,则 $a, b$ 共线当且仅当 #v(10pt)
- $lambda_a autoVec3(a) + lambda_b autoVec3(b) = 0 space (lambda_a, lambda_b "不全为零")$ #v(10pt)
- $mat(delim: "|",
a_1 , a_2 ;
b_1 , b_2 ;
)
= mat(delim: "|",
a_2 , a_3 ;
b_2 , b_3 ;
) =
mat(delim: "|",
a_3 , a_1 ;
b_3 , b_1 ;
) = 0$ #v(10pt)
- $mat(
a_1, b_1;
a_2, b_2;
a_3, b_3;
) $的秩为 $1$
]
#corollary[][
空间中三点 $A, B, C$ 三点共线当且仅当
- $arrow(A C), arrow(B C)$ 共线
- 对空间中任意一点 $O$,$existsST(t, arrow(O C) = t arrow(O A) + (1-t) arrow(O B))$ \
注:当 $O$ 不在这条直线上时,上述分解是唯一的
]
#definition[简单比][
设 $A, B, C$ 为共线三点,定义简单比:
$
(A, B; C) = arrow(A C)/ arrow(C B)
$
]<简单比>
#proposition[][
- $(A, B; C) = 1/(B, A; C)$
- $(A, B; C) + (A, C; B) = -1$
]
== 向量乘积
(历史上来讲,事实上比四元数还要晚)\
设 $a, b in EE^3$ \
定义:内积/外积/垂直......\
正交分解:$a = (a dot b) / (|b|^2) b + a_b$,其中 $a_b dot b = 0$,此分解成立且唯一
内积的性质:
- 交换性
- $a dot a = a^2$
- 结合性:$(lambda a) dot b = lambda (a dot b)$
- 线性性:$(lambda a + mu b) dot c = lambda a dot c + mu a dot c$
只证最后一个,不妨假设 $lambda = mu = 1$\
此时有
$
a = (a dot c) / (|c|^2) c + a_c\
b = (b dot c) / (|b|^2) c + b_c
$
代入上式结合点积等于投影立得
单位正交基:......
外积/向量积/叉乘:......
性质:
+ 反交换律
+ 数乘结合律
+ 分配律
在 $n$ 维空间中,也可定义 $n-1$ 元算子,但是不构成代数,因此不便于使用。由于八元数也可除,在 $7$ 维空间也可定义外积,但乘法不结合。因此类似外积的运算仅在 3 维空间中使用,在更一般的情况下,使用外积的代数结构称为外代数
#proposition[拉格朗日公式][
$
a times (b times c) = (a dot c) b - (a dot b) c \
(a times b) times c = (a dot c) b - (b dot c) a
$
换言之,向量积不结合
]
#proof[
(假定所有向量线性无关)\
注意到 $a times (b times c),b,c$ 均与 $b times c$ 正交,因此待定系数:
$
a times (b times c) = lambda b + mu c
$
两边与 $a$ 点乘:
$
0 = lambda a dot b + mu a dot c
$
]
#proposition[Jacobi恒等式][
$
a times (b times c) + b times (c times a) + c times (a times b) = 0
$
]
在几何上,它表示三角形 $A B C$ 的三条高线交于一点
#definition[混合积/体积][
$
(a, b, c) = (a times b) dot c
$
在单位正交基下,它就是 $autoMat3(delim: "|",a , b, c)$
]
#proposition[][
混合积有如下性质:
- 反对称性: $(a, b, c) = -(b, a, c)$
- 轮换对称性:$(a, b, c) = (c, a, b) = (b, c, a)$
- 线性性: $(lambda a + mu b, c, d) = lambda (a, c, d) + mu (b, c, d)$
]
#proposition[][
混合积在几何上等于平行六面体的有向体积
]
#proposition[][
$(a times b) dot (c times d) = mat(delim: "|", a times b, b times c ; c times d, d times a ; ) = (a times c) dot (b times d) - (a times d) dot (b times c)$
]
#corollary[][
$|a times b|^2 = |a|^2 |b|^2 - (a dot b)^2$
]
== 应用:球面几何
考虑三维空间中球面(称为二维球面 $S_2$)。设球面上两点 $P, Q$ 不是对径点,则它们确定了唯一一个大圆,这个大圆弧称为(球面几何的)直线或者测地线。在下面的讨论中,不妨假设球面是单位球面。
#proposition[][
任何两个不同的直线交于两点,任何三个不同直线组成球面三角形(事实上是两个三角形,但它们是全等的)。显然三角形的边长等于对应球心角的角度。
]
#definition[角度][
两直线的角度定义为测地线相交处切线的夹角。
]
#theorem[面积][
球面三角形 $A B C$ 的面积为:
$
A + B + C - pi
$
其中 $A, B, C$ 指三个顶角的角度。\
]
#proof[
取 $A, B, C$ 对径点为 $A', B', C'$\
$l_(A C), l_(A B)$ 分球面为四部分。\
包含 $B C$ 与 $B' C$ 的两部分面积为:
$
A / (2 pi) dot 2 dot 4 pi = 4 A
$
$l_(A B), l_(B C)$ 分球面为四部分,包含 $A C$ 与 $A' C'$ 的两部分面积为:$4 B$\
$l_(A C), l_(B C)$ 分球面为四部分,包含 $A B$ 与 $A' B'$ 的两部分面积为:$4 C$\
这六个部分覆盖三角形 $A B C$ 与 $A' B' C'$ 各三次。覆盖其余部分各一次。由此:
$
4 A + 4 B + 4 C = 4 pi + 4 S
$
注:这里假定了球面上面积也是有限可加,等距不变的
]
#corollary[][
- 球面三角形的内角和大于 $pi$
- 球面上不存在相似三角形,从而三个角唯一确定了三角形
]
#theorem[球面几何三角公式][
设 $A B C$ 是球面三角形,角 $A, B, C$ 所对边长度为 $a, b, c$,则有:
- 正弦定理:
$
sin(a) / sin(A) = sin(b) / sin(B) = sin(c) / sin(C)
$
- (第一)余弦定理:(长度决定角度)
$
cos(c) = cos(a)cos(b) + sin(a)sin(b)cos(C)
$
注:对足够小的 $a, b, c$,这给出了平面的余弦定理
- 第二余弦定理:(角度决定长度)
$
cos(C) = -cos(A) cos(B) + sin(A) sin(B) cos(c)
$
]<球面几何正余弦>
#proof[
记 $arrow(O A), arrow(O B), arrow(O C) = alpha, beta, gamma$,则 $a, b, c$ 分别是三组向量的夹角。另一方面,$A, B, C$ 分别是三组大圆面的平面夹角。换言之:
$
A = generatedBy(alpha times beta\, gamma times alpha)\
B = generatedBy(beta times gamma\, alpha times beta)\
C = generatedBy(gamma times alpha\, beta times gamma)
$
由拉格朗日公式:
$
(alpha times beta) times (alpha times gamma) = ((alpha times beta) dot gamma) alpha - ((alpha times beta) dot alpha) gamma = ((alpha times beta) dot gamma) alpha = [alpha, beta, gamma] alpha
$
又由$|alpha times beta| = 1 dot 1 dot sin(c) ......$,这表明:
$
sin(c)sin(b)sin(A) = [alpha, beta, gamma]
$
轮换即得正弦定理。\
又:
$
(alpha times beta) dot (alpha times gamma) = (alpha dot alpha) (beta dot gamma) - (alpha dot beta) (alpha dot gamma)
\ => sin(c)sin(b)cos(A) = cos(a) - cos(b)cos(c)
$
这就是余弦定理。\
为了证明第二余弦定理,取三角形 $A' B' C'$ 为三角形 $A B C$ 的极三角形。也即,取:
$
alpha' = (beta times gamma) / (|beta times gamma|)\
beta' = (gamma times alpha) / (|gamma times alpha|)\
gamma' = (alpha times beta) / (|alpha times beta|)
$断言:
$
a' = pi - A, b' = pi - B, c' = pi - C
$
事实上,在前式中取 $cos$ 立得。\
另一方面:
$
alpha' times beta' = ([gamma, alpha, beta] gamma) / (sin(a) sin(b))\
alpha' times gamma' = - ([gamma, alpha, beta] beta) / (sin(a) sin(c))
$
从而
$
cos(A') = generatedBy(alpha' times beta'\, gamma' times alpha') = - gamma dot beta => A' = pi - a("注意到" alpha, beta "均为单位向量")
$
在极三角形 $A' B' C'$ 中,代入余弦定理即得原三角形的第二余弦定理。
]
= 平面二次曲线的分类
#definition[二次曲线][
二次曲线是笛卡尔坐标系上满足二次方程:
$
Tau: a x^2 + b x y + c y^2 + d x + e y + f = 0
$
的点的集合。
]
对于一般的方程,为了研究图形,可以考虑通过移动和旋转坐标轴的方式使得方程简化。
== 仿射坐标系
称 $(O, e_1, e_2)$ 为一个仿射标架,其中 $O$ 是原点,$e_1, e_2$ 是两个不共线的向量。\
在此标架下,任意向量可被唯一表为:
$
(e_1, e_2) vec(x, y)
$
另取一仿射标架 $(O', e'_1, e'_2)$,并设:
$
(e'_1, e'_2) = (e_1, e_2)A
$
则有:
$
V =(e_1, e_2)vec(x, y) = (e'_1, e'_2)vec(x', y') = (e_1, e_2)A vec(x', y')\
=> vec(x, y) = A vec(x', y')
$
这称为向量的坐标变换。\
对于平面上的点,完整的坐标变换公式为:
$
(e_1, e_2)vec(x, y) = O P = O O' + O' P
\ = O O' + (e'_1, e'_2)vec(x', y')
\ = O O' + (e_1, e_2)A vec(x', y')
\ => vec(x, y) = A vec(x', y') + "cor"(O O')
$
也可将其统一为:
$
vec(x, y, 1) = mat(A, beta;0, 1) vec(x', y', 1)
$
其中 $beta = "cor"(O O')$。\
显然它可以分解为两部分:移轴(保持 $e_1, e_2$ 不变)和旋转(保持原点不变)。就方程而言,移轴可以消去一次项,而转轴可以消去交叉项。\
== 单位正交坐标系
在单位正交系中我们也可以进行坐标系变换,但我们希望保持正交性。\
令 $(O, e_1, e_2)$ 是右手单位正交坐标系。我们希望它经过变换后得到 $(O', e'_1, e'_2)$ 仍是单位正交坐标系。\
#proposition[][
设 $(e'_1, e'_2) = A$,则 $(O', e'_1, e'_2)$ 仍是右手单位正交坐标系当且仅当 $A$ 是正交矩阵且行列式为 $1$。
]
#proof[
在三维空间中,取右手单位正交框架 $(O, e_1, e_2, e_3)$。\
我们希望 $(O, e'_1, e'_2, e_3)$ 也是右手单位正交框架,也即:
$
vec(e'_1^T, e'_2^T, e_3^T)(e'_1, e'_2, e_3) = I\
[e'_1, e'_2, e_3] = |e'_1, e'_2, e_3| = 1 \
$
前式等价于:
$
vec(e_1^T, e_2^T, e_3^T)mat(A^T, 0;0, 1)mat(A, 0;0, 1)(e_1, e_2, e_3) = I
\ <=> A^T A = I
$
后式等价于:
$
1 = mat(delim: "|", A, 0;0,1)|e_1, e_2, e_3| = |A|
$
这就证明了命题
]
#corollary[][
二维正交矩阵均可以表示为:
$
mat(cos theta, -sin theta ;sin theta , cos theta )
$
进而,平面上两右手单位正交系间的坐标变换可以表示为:
$
vec(x, y) = mat(cos theta, -sin theta ;sin theta , cos theta )vec(x', y') + vec(x_0, y_0)
$
其中,$vec(x_0, y_0)$ 是 $O'$ 在原坐标系下的坐标,$theta$ 表示由原坐标轴逆时针旋转到新坐标轴经过的角度。
]
== 标准二次曲线
+ 椭圆:$x^2/a^2 + y^2/b^2 = 1$
+ 双曲线:$x^2/a^2 - y^2/b^2 = 1$
+ 抛物线:$y^2 = 2 p x$
+ $(a_1 x + b_1 y + c_1)(a_2 x + b_2 y + c_2) = 0$ 双直线
+ $(x - p)^2 + (y - q)^2 = 0$ 单点
+ $(x - p)^2 + (y - q)^2 = -1$ 无解
显然这些曲线在正交变换下不改变。\
问题:如何确定标准型?
- 考虑移轴、转轴
- 考虑不同变换群下的不变量
== 正交变换不变量
二次方程可以记作一个矩阵,从而二次曲线可以写作:
$
x^T A x = 0 ; A = mat(A_1, alpha; alpha^T, c)
$
一般的正交变换可以用矩阵:
$
Q := mat(B, beta;0, 1)
$
替代,其中 $B$ 为正交矩阵;\
这相当于在一般的合同变换中,在 $1, 2$ 行/列 与 $3$ 行列之间只允许高行列加减低行列,反之不可。\
在此变换下 $A$ 变为
$
Q^T A Q = mat(B^T A_1 , B^T alpha; beta^T A_1 + alpha^T , beta^T alpha + c)Q
\ = mat(B^T A_1 B, B^T A_1 beta + B^T alpha;beta^T A_1 B + alpha^T B, beta^T A_1 beta + alpha^T beta + c)
$
不难发现:
- $|A| = |Q^T A Q| := I_3$
- $|A_1| = |Q^T A_1 Q| := I_2$
- $tr(A_1) = tr(B^T A_1 B) := I_1$
是三个移轴、转轴变换下的不变量\
注:$tr(A)$ 未必是不变量,因为 $tr(A) = tr(Q^T A Q)$,但 $Q$ 未必正交。
有了不变量,我们就可以做如下分类:
+ $I_2 > 0$ 椭圆型:
$
mat(lambda_1, 0, 0;0, lambda_2, 0;0, 0, e)
$
- $I_1 I_3 < 0$ 椭圆
- $I_1 I_3 > 0$ 空集
- $I_1 I_3 = 0$
- $I_1 = 0$,显然不可能
- $I_3 = 0$,单点
+ $I_2 < 0$ 双曲型:
$
mat(lambda_1, 0, 0;0, - lambda_2, 0;0, 0, e)
$
- $I_3 != 0$ 双曲线
- $I_3 = 0$ (相交的)双直线
+ $I_2 = 0$ 抛物线型:
$
mat(lambda_1, 0, 0;0, 0, d;0, d, e)
$
- $I_3 != 0$ 抛物线
- $I_3 = 0$:
此时 $A$ 有以下形态:
+ $I_1 = lambda_1 != 0$
$
mat(lambda_1, 0, 0;0, 0, 0;0, 0, e)
$
- $lambda_1 e > 0$ 空集(或称之为平行虚直线)
- $lambda_1 e < 0$ 两条平行直线
+ $I_1 = lambda_1 = 0$
$
mat(0, 0, 0;0, 0, d;0, d, e)
$
退化为任意一次曲线
对于剩余情形,引入半不变量:
$A$ 的二阶主子式的和记作 $k_1$
#proposition[][
若 $I_2 = I_3 = 0$,则 $k_1$ 在转轴、移轴下不变。
]
== 圆锥曲线的几何性质
圆锥曲线在古典几何中有着很重要的位置,早在古希腊便有很多研究。\
在几何上,圆锥曲线可以统一定义为:
- 用任意平面截取圆锥,得到的曲线称为圆锥曲线
- 在平面上,到定点的距离与到定直线的距离之比为定值的点的轨迹称为圆锥曲线,定点、定直线、比例分别为焦点、准线、离心率
显然,这些性质应该不依赖于直角坐标系的选取。事实上,它们都是度量特征(在正交变换下不变)\
与之相对的,曲线分类、中心、对称轴、开口方向等性质在任意仿射变换下不变。\
研究几何性质往往从直线与圆锥曲线的相交情况出发:\
设圆锥曲线方程为 $X^T A X = 0; A = mat(A_1, alpha; alpha^T, c)$。
另设直线方程为:$X_0 + lambda beta; beta = vec(u, v, 0)$\
代入:
$
(X_0 + lambda beta)^T A (X_0 + lambda beta) \
= X_0^T A X_0 + 2 lambda X_0^T A beta + lambda^2 beta^T A beta = 0
$
记:
- $beta^T A beta = beta^T A_1 beta$ 为 $phi(u, v)$
- $X_0^T A beta = (x, y, 1) mat(A_1, alpha; alpha^T, c) vec(u, v, 0) = ((x, y) A_1 + alpha^T, (x, y)alpha + c)vec(u, v, 0) = ((x, y) A_1 + alpha^T)vec(u, v) \ := u F_1(x, y) + v F_2(x, y)
$
- 容易验证 $F_1(x, y) = (delta F(x, y))/(delta x), F_2(x, y) = (delta F(x, y))/(delta y)$
=== 中心
下面的讨论中,假设 $A_1 != 0$,从而保证有无穷多组 $beta$ 使得上述方程是二次方程\
在上面的方程中,假设 $X_0$ 恰好是中心,则由韦达定理,任意联立方程的一次项均为零,即有:
$
forall beta in R^2, u F_1(x, y) + v F_2(x, y) <=> F_1(x, y) = F_2(x, y) = 0 <=> (x, y, 1) vec(A_1 ,alpha^T) = 0
$
容易验证这是充要的。
- 椭圆型、双曲线型一定有唯一中心:$A_1$ 此时可逆故解显然存在且唯一。(对于一些退化情况,可能是广义的中心)
- 抛物线型中:
- 抛物线无中心
- 一次曲线的退化情况不能如此讨论
- 其余情况均有一条直线作为中心的集合
=== 渐进方向
#definition[渐进方向][
若 $phi(u, v) = 0$,则称 $vec(u, v)$ 是一个渐进方向。
]
这可以理解为直线与圆锥曲线将在无穷远处相交。(在方程两边除以 $t$,我们发现 $1/t = 0$ 将成为一个伪解)\
显然渐进方向取决于 $A_1$ 的正惯性指数。\
- 若 $A_1$ 正定或负定,也即椭圆型,则 $phi(u, v) > 0$,不存在渐进方向
- 若 $|A_1| = 0$,也即抛物线型,此时恰有一个渐进方向也即零特征值的特征向量
- 若 $A_1$ 特征值一正一负,此时 $(u, v)$ 是渐进方向当且仅当:
$
lambda_1 u^2 + lambda_2 v^2 = 0
$
显然它可以唯一分解为两个渐进方向
#proposition[][
如此定义的渐近线和分析意义的渐近线是等价的。
]
#proposition[][
- 椭圆型曲线无渐近线
- 双曲线型恰由两个渐进方向
- 抛物线型恰有一个渐进方向
- 过双曲线中心的渐近线与双曲线不相交
- 抛物线型曲线的任意渐近线都与抛物线相交
]
#proposition[][
中心在曲线上意味着有56
]
对于抛物线,我们需要判断渐进方向的哪一侧是抛物线的开口方向。
#lemma[][
$X$ 在抛物线的内部当且仅当:
$
(X^T A X) I_1 < 0
$
]
#proposition[][
$(u, v)$ 是开口方向当且仅当:
$
I_1 (u D + v E) > 0
$
]
== 共轭直径
对于圆而言,一簇平行直线与圆的交点的中点在一条直线上。对椭圆同样也有类似性质。一般的:
#theorem[][
设 $A$ 是二次曲线,且 $(u, v)$ 不是渐进方向,则所有平行于 $(u, v)$ 的直线与 $A$ 的交点的中点在一条直线上,该直线的方向称为 $(u, v)$ 的共轭方向。
]
#proof[
设 $beta = vec(u, v, 0)$\
在前述的联立方程中,可得:
$
(X_1 + X_2) / 2 &= X_0 + (t_1 + t_2)/2 beta
\ &= X_0 - (X_0^T A beta) / (beta^T A_1 beta) beta
\ &= X_0 - (beta^T A X_0) / (beta^T A beta) beta
$
注意到:
$
(beta^T A) (X_1 + X_2) / 2 = beta^T A X_0 - (beta^T A X_0) / (beta^T A beta) beta^T A beta = 0
$
这意味着中点在直线:
$
(beta^T A) X = 0
$
上,除非:
$
A beta = 0
$
此时 $beta$ 是渐进方向。\
显然这是一条过中心(如果有)的直线。\
]
#proposition[][
- 二次曲线的共轭方向(只要不涉及渐进方向)互相共轭,称之为一对共轭直径。\
$gamma$ 是 $beta$ 的共轭方向 $<=> gamma^T A alpha = 0 <=> alpha^T A gamma = 0 <=>$ $beta$ 是 $gamma$ 的共轭方向
- 对于非抛物线型曲线,不会出现非渐进方向的共轭方向是渐进方向,但抛物线型有可能。
注意到:
$
beta^T A_1 alpha = alpha^T A_1 alpha = 0 "且" alpha, beta "线性无关" => A_1 alpha = 0 => |A_1| = 0
$
]
== 切线
#definition[][
若直线与二次曲线只有一个交点且不是渐进方向上,则称直线与二次曲线相切。
]
此定义相当于对切线做以微扰便可得到正常相交的直线。\
#proposition[][
对任意二次曲线上的点 $X_0$,只要它不是中心,便可得到唯一的切线。\
只需解:
$
beta^T A X_0 = u F_1(x_0, y_0) + v F_2(x_0, y_0) = 0
$
对应的 $beta$ 即可
]
#proposition[][
对任意方向,只要它不是渐进方向,便可按以下方法得到这个方向上的切线(可能没有或一、两个)。\
设给定方向 $beta = vec(u, v, 0)$,只需解
$
X_0^T A X_0 = 0\
beta^T A X_0 = 0\
$
即可。\
更进一步,若曲线有中心 $O$,则二式表明 $X_0 - O$ 就是 $beta$ 的共轭方向。因此解的数量等价于过中心沿此方向与曲线相交的点的数量。
]
== 圆锥曲线的度量特征
=== 对称轴
容易发现,若一个方向的直线恰与其垂直,则直线即为圆锥曲线对称轴。这等价于 $A_1$ 的特征向量所对应的方向。\
#proposition[][
- 对于圆,每个方向都是一个对称轴
- 对于椭圆、双曲线,恰有两个特征向量,对应两个对称轴,也称其为主方向
- 对于抛物线,渐进方向也即 0 特征值对应的特征向量是唯一的对称轴
]
有了对称轴后,容易得到椭圆、双曲线的中心以及抛物线的顶点。\
#example[][
$
Q = mat(8, 4, -3;4, 2, -4;-3, -4, 1);
$
计算得:
$
I_1 = 10\
I_2 = 0\
I_3 = -50
$
从而它是抛物线。
$A_1$ 的特征向量分别为:
$
0 -> vec(1, -2)\
10 -> vec(2, 1)\
$
从而对称轴方程应为:
$
(2, 1)mat(8, 4, -3;4, 2, -4;) vec(x, y, 1) = 0\
<=> 20 x + 10 y - 10 = 0
<=> 2 x + y - 1 = 0
$
进而联立解得顶点即可。
$
beta := vec(2, 1, -2)\
beta_0 := vec(2, 1, 0)\
beta = beta_0^T A\
beta_0 A X = 0\
X^T A X = 0
$
]
= 空间解析几何
== 平面方程
空间中一个平面的方程为一个线性方程:
$
A x + B y + C = 0
$
它可以由以下事实给出:
#lemma[][
空间中四点 $autoVec3(x), autoVec3(a), autoVec3(b), autoVec3(b)$ 共面当且仅当:
$
autoMat3(delim: "|", x, b, c) = autoMat3(delim: "|", a, b, c)
$
]
== 直线方程
=== 标准方程
空间中直线可以写作:
$
X = X_0 + t vec(u, v, w) \
<=> (x-x_0)/u = (y-y_0)/v = (z-z_0)/w
$
这称为直线的标准方程
=== 相交方程
空间中两个不平行平面唯一给定一个交线,从而直线可表示为:
$
cases(
pi_1 X = D_1,
pi_2 X = D_2
)
$
显然交线的方向向量即为 $pi_1 times pi_2$,再任取一个交点即可得到直线的标准方程。
== 相交关系
两平面间的相交情况显然就是线性方程组:
$
vec(pi_1^T, pi_2^T) X = vec(D_1, D_2)
$
的解集。\
两直线间的相交可以转换为线性方程组:
$
X_1 +t_1 V_1 = X_2 + t_2 V_2\
<=> (V_1, V_2) X = X_2 - X_1
$
记 $"rank"(V_1, V_2) = r_1, "rank"(V_1, V_2, X_2 - X_1) = r_2$
- 两直线异面 $<=> r_2 = 3$
- 两直线相交 $<=> r_2 = r_1 = 2$
- 两直线平行 $<=> r_2 = 1, r_3 = 2$
- 两直线重合 $<=> r_2 = r_1 = 1$
直线与平面间的相交关系可以转换为线性方程组:
$
vec(pi_1^T, pi_2^T, pi_3^T) X = autoVec3(D)
$
或者:
$
cases(
pi^T X = D,
X = X_0 + t V
)
<=> pi^T (X_0 + t V) = D\
<=> pi^T V t = D - pi^T X_0
$
== 度量关系
=== 平行平面距离
设两平行平面:
$
pi^T X = D_1\
pi^T X = D_2
$
则它们的距离为:
$
(|pi^T (X_1 - X_2)|) / (|pi|) = (|D_1 - D_2|) / (|pi|)
$
=== 点到平面距离
设平面:
$
pi^T X = D
$
则点 $X_0$ 到平面的距离为:
$
(|pi^T (X_0 - X)|) / (|pi|) = (|D - pi^T X_0|) / (|pi|)
$
=== 点到直线距离
设直线:
$
X = X_0 + t V
$
对 $X-X_0$ 关于 $v$ 做正交分解:
$
X - X_0 = k v + v'
$
其中 $|v'|$ 就是我们想要的,我们有:
$
(X_1 - X_0) times V = v' times V\
|(X_1 - X_0) times V| = |v' times V| = |v'| |V|\
$
则点 $X_1$ 到直线的距离为:
$
(|(X_1 - X_0) times V|) / (|V|)
$
=== 直线间距离
平行直线间距离就是任意一点与另一直线的距离。\
异面直线的距离
= 二次曲面
对于曲面,可以分成几种简单的类型
== 旋转面
#definition[旋转面][
旋转面是由一条曲线绕一条轴旋转一周得到的曲面。\
称其中的曲线为母线,轴为准线。
]
#proposition[][
曲线:$f(y, z) = 0, x = 0$ 绕 $z$ 轴旋转一周得到的曲面方程为:
$
f(sqrt(x^2 + y^2), z) = 0
$
]
#proposition[][
设 $X_0 + t v$ 为轴,则 $M$ 在旋转面上当且仅当:
$
exists M_0 "在曲线上": (|(M_0 - X_0) times v|)/ (|v|) = (|(M - X_0) times v|)/ (|v|)
$
]
== 柱面
#definition[][
柱面是由一条曲线沿一个方向平移得到的曲面。\
]
#proposition[][
设 $tau$ 为准线,$v$ 为(母线)方向,则 $X$ 在锥面上当且仅当:
$
exists X_0 in tau: (X - X_0) times v = 0
$
]
== 锥面
#definition[][
一组过同一点 $M$ 的直线构成锥面\
称:
- $M$ 为顶点
- 一组直线中任意一条称为直母线
- 锥面上不过顶点但与所有母线相交的曲线为准线
]
#proposition[][
设 $M$ 为顶点,$tau$ 为准线,则 $X$ 在锥面上当且仅当:
$
exists X_0 in tau: (X_0 - M) times (X - M) = 0
$
]
== 直纹面
#definition[][
空间中曲面 $Sigma$ 称为直纹面,若对任意 $P in Sigma$,均存在过 $P$ 的直线 $l$ 使得 $l subset Sigma$
]
#proposition[][
锥面、柱面都是直纹面
]
二次曲线中,大部分面是否是直纹面都是直观的(显然有界曲面不会是直纹面),但双曲抛物面有有趣的结论:
#theorem[][
双曲抛物面是直纹面
]
#proof[
注意到:
$
2 z = x^2/a^2 - y^2/b^2 = (x/a + y/b)(x/a - y/b)
$
因此对曲面上点 $P$,存在某个 $c$ 使得:
$
cases(
x/a + y/b = c,
x/a - y/b = (2z) / c
)
$
或者:
$
cases(
x/a + y/b = (2z) /c,
x/a - y/b = c
)
$
对应的点都在曲面上,且它是一条过 $P$ 的直线。\
]
在上面的证明中,我们得到了两族不同的直母线。
#theorem[][
单叶双曲面(马鞍面)是直纹面
]
#proof[
$
x^2/a^2 + y^2/b^2 - z^2/c^2 = 1\
<=> (x/a + z/c)(x/a -z/c) = (1 - y/b)(1 + y/b)
$
从而对某个 $c$:
$
cases(
x/a + z/c = (1 + y/b)c,
x/a - z/c = (1 - y/b)/c
)
$
或:
$
cases(
x/a + z/c = (1 - y/b)c,
x/a - z/c = (1 + y/b)/c
)
$
之后是类似的。
]
== 二次曲面的分类
一般的二次曲面可以写作:
$
(x, y, z, 1) mat(A_1, alpha; alpha^T, c) vec(x, y, z, 1) = 0
$
用类似讨论二次曲线的方法,可以得到二次曲面的标准方程以及不变量
一般的三维空间正交变换矩阵可以写作:
$
Q = mat(Q, beta; 0, 1)
$
从而:
$
Q^T A Q = mat(Q, 0; beta^T, 1) mat(A_1, alpha; alpha^T, c)mat(Q, beta; 0, 1) = mat(Q^T A_1 Q, *; *, *)
$
由对称矩阵的性质,断言 $A_1$ 一定可以对角化。\
可以写出以下不变量:
- $I_1 = tr(A_1)$
- $I_2 = K_2(A_1)$ 是 $A_1$ 的二阶主子式之和
- $I_3 = |A_1|$
- $I_4 = |A|$
同样也可以从同时行列变化的角度,在 4 行/列与 1、2、3 行/列之间只允许高行列加减低行列,反之不可。\
因此分类可以直接从标准型:
$
mat(lambda_1, 0, 0, a;0, lambda_2, 0, b;0, 0, lambda_3, c;a, b, c, d)
$
开始。\
+ $I_3 != 0$,此时标准型为:
$
mat(lambda_1, 0, 0, 0;0, lambda_2, 0, 0;0, 0, lambda_3, 0;0, 0, 0, d)
$
由于我们得到了三个特征值,因此可以不妨设 $I_3 > 0$
+ $I_1, I_2 > 0$,也即 $lambda_i > 0$
- $I_4 < 0$ 椭球面:$x^2/a^2 + y^2/b^2 +z^2/c^2 = 1$
- $I_4 > 0$ 空集
- $I_4 = 0$ 单点
+ 其他情况均有其中两个特征值同号,不妨设 $lambda_1, lambda_2 > 0, lambda_3 < 0$
- $I_4 < 0$:单叶双曲面:$x^2/a^2 + y^2/b^2 -z^2/c^2 = 1$
- $I_4 > 0$:双叶双曲面:$x^2/a^2 + y^2/b^2 -z^2/c^2 = -1$
- $I_4 = 0$:椭圆锥面:$x^2/a^2 + y^2/b^2 -z^2/c^2 = 0$
+ $I_3 =0, I_2 != 0$,此时标准型为:
$
mat(lambda_1, 0, 0, 0;0, lambda_2, 0, 0;0, 0, 0, c;0, 0, c, d)
$
有:
$
I_4 = -lambda_1 lambda_2 c^2
$
+ $I_4 != 0 <=> c != 0$,此时可消去 $d$
- $I_2 = lambda_1 lambda_2 > 0$:椭圆抛物面:$x^2/a^2 + y^2/b^2 = z$
- $I_2 = lambda_1 lambda_2 < 0$:双曲抛物面:$x^2/a^2 - y^2/b^2 = z$
+ $I_4 = 0 <=> c = 0$
- $d != 0$
- $I_2 = lambda_1 lambda_2 > 0$:椭圆柱面(或空集):$x^2/a^2 + y^2/b^2 = d$
- $I_2 = lambda_1 lambda_2 < 0$:双曲柱面:$x^2/a^2 - y^2/b^2 = d$
- $d = 0$
- $I_2 = lambda_1 lambda_2 > 0$:退化为一条直线:$x^2/a^2 + y^2/b^2 = 0$
- $I_2 = lambda_1 lambda_2 < 0$:两个相交平面:$x^2/a^2 - y^2/b^2 = 0$
+ $I_3 =0, I_2 = 0, I_1 != 0$,此时标准型为:
$
mat(lambda_1, 0, 0, 0;0, 0, 0, b;0, 0, 0, c;0, b, c, d)
$
+ $b = c = 0$:
- $lambda_1 d > 0$:空集
- $lambda_1 d < 0$:单点
- $lambda_1 d = 0$:一个平面(两个重合平面)
+ $b, c$ 有且只有一个为零,不妨设 $b!= 0, c = 0$,$d$ 也可消去
- 抛物柱面:$x^2/a^2 = y$
+ $I_3 =0, I_2 = 0, I_1 = 0$,此时标准型为:
$
mat(0, 0, 0, a;0, 0, 0, b;0, 0, 0, c;a, b, c, d)
$
已经退化为一次曲面
至此,我们圆满完成了二次曲面的分类
= 变换
== 保距变换
#definition[保距变换][
称几何空间上的变换 $sigma$ 是保距变换,如果:
+ $sigma$ 可逆
+ $forall x, y in EE$,有:
$
d(x, y) = d(sigma(x), sigma(y))
$
]
#proposition[][
保距变换的逆映射和复合仍是保距变换,进而几何空间上的保距变换构成一个群。
]
$EE^3$ 中保距变换的全体记作 $"Isom"(EE^3)$。\
#example[][
以下是典型的保距变换:
+ 平移
+ 旋转
+ 关于点、线的反射
+ 滑反射:反射后再平移
+ 旋进:绕一点旋转一定角度后再平移,例如地球上某点相对太阳
]
#lemma[][
+ 保距变换保持平行四边形
+ 保距变换保持线段夹角不变
+ 保距变换保持向量,也即点上的保距变换可以诱导出良定义的向量上的保距变换
+ 保距变换保持向量内积不变
+ 保距变换保持向量外积不变
+ 保距变换对向量的作用是线性的
]
#proof[
1 由保距性是显然的。\
对于 2,显然 $A B C$ 与 $sigma(A) sigma(B) sigma(C)$ 全等,因此结论易得。\
3 中,只需定义自然诱导的向量保距变换是良定义的,为此只需要证明相等且平行的线段的像依然相等且平行,这由 1 显然。\
对于 4、5,由 1,2 结合内积定义显然(注意保持夹角意味着保持垂直)\
对于 6,考虑设 $e_1, e_2, e_3$ 是一组单位正交基,它们的像分别为 $v_1, v_2, v_3$。
由前面的命题,$v_1, v_2, v_3$ 也是单位正交基,因此设:
$
v := sum_i lambda_i e_i\
sigma(v) = sum_i lambda'_i v_i
$
由保持内积知 $lambda_i = v dot e_i = v dot v_i = lambda'_i$,这意味着:
$
sigma(v) = sigma(sum_i lambda_i e_i) = sum_i lambda_i sigma(e_i)
$
这就证明了 $sigma$ 在向量上的线性性。
]
#proposition[][
$EE^3$ 中不共面四点唯一决定一个保距变换
]
#proof[
设 $P_1, P_2, P_3, P_4$ 的像分别为 $Q_1, Q_2, Q_3, Q_4$。\
显然 $overline(P_1 P_2), overline(P_1 P_3), overline(P_1 P_4)$ / $overline(Q_1 Q_2), overline(Q_1 Q_3), overline(Q_1 Q_4)$ 是两组基。\
设 $v = sum_i lambda_i overline(P_1 P_i)$,由线性性,立得:
$
sigma(overline(v P_1)) = sum_i lambda_i overline(Q_1 Q_i)
$
这就表明任何点的像已经确定,证毕。
]
#proposition[][
$EE^3$ 中任何保距映射可以由四个关于平面的反射复合而成
]
#proof[
在上面的命题中,取 $A, B, C, D$ 四点。先取 $A$ 与 $sigma(A)$ 的中垂面,构造 $pi_1$ 是关于该平面的反射,显有 $pi_1(A) = sigma(A)$
再取 $B, pi_2(sigma(B)$) 的中垂面。注意到由保距变换,$|A B| = |A pi_2(sigma(B)|$,因此点 $A$ 在这个中垂面上。依次取得 $pi_2$,$pi_3$,$pi_4$,则有:
$
sigma = pi_4 pi_3 pi_2 pi_1
$
]
#proposition[][
空间中保距变换若有不动点,则它是关于某条直线的对称
]
#proposition[][
一般的保距变换是关于某直线对称和平移的复合
]
#proposition[][
以下保距变换的性质是与坐标无关的
- 旋转角度
- 平移距离
]
#proposition[][
平面上的保定向(行列式大于零)的保距变换只有平移和旋转
平面中的保距变换是平移,旋转,反射,滑反射其一。
]
#proof[
- 若保距变换保定向,则它没有实特征值,因此它是旋转或平移
- 若保距变换有不动点,则它等价于向量的正交变换,而二维的正交变换只有反射和旋转
- 若无不动点,则它等价于保持原点不动的保距变换与平移的复合,从而要么是平移,要么是滑反射(注:平移和旋转的复合有不动点)
]
== 仿射变换
#definition[仿射变换][
若几何空间上的可逆变换 $sigma$ 保持直线不变,则称 $sigma$ 是仿射变换。
]
#proposition[][
由保距变换在向量上的作用的线性知,保距变换是仿射变换。
]
#example[][
典型的仿射变换有:
- 保距变换
- 正压缩:$lambda_1 l + lambda_2 l' -> lambda_1 l + k lambda_2 l'$
- 错切:$lambda_1 l + lambda_2 alpha_2 -> lambda_1 l + (lambda_2 + k lambda_1) alpha_2$
- 斜压缩:$lambda_1 l + lambda_2 u -> lambda_1 l + k lambda_2 u$
]
#example[][
向量的线性变换可以自然诱导出仿射变换,因此以下矩阵也是仿射变换:
- $mat(0, 1;-1, 0)$,这是一个旋转+反射
- $mat(1, 1;1, 2)$,这是两个错切
- $mat(1, -1;1, 0)$,这是一个错切
]
#proposition[][
二阶线性变换群恰为:
$
generatedBy(S\, R| S^4 = R^6 = 1\, S^2 = R^3)
$
其中 $S = mat(1, 1;0, 1), R = mat(1, 0;1, 1)$
]
#proposition[][
以 $l$ 为轴的斜压缩是以 $l$ 为轴的错切和正压缩的复合
]
#lemma[][
+ 仿射变换保持直线相交、平行
+ 仿射变换保持平行四边形、平行六面体
+ 仿射变换保持平面
+ 仿射变换保持向量,也即点上的仿射变换可以诱导出良定义的向量上的仿射变换
]
#proof[
1: 由保持直线知,两个直线的交点的像应该同时在两个直线的像上,因此保持直线的相交、平行。\
2:由保持平行容易得到
3:注意到平面由两条相交直线决定,只需证明任何在平面上,过初始两直线交点的直线都在新平面上即可\
4 由前面命题是显然的。
]
#theorem[][
仿射变换保持分比 $<=>$ 仿射变换在向量上的作用是线性的
]
#proof[
这个证明是很不平凡的,我们的目标是证明:仿射变换诱导的向量变换是可逆的线性变换。\
良定义性前面已知,可逆性是容易证明的,关键是证明保持线性运算。\
其中,保持加法由保持平行四边形是平凡的,下面证明保持数乘
+ 由保持加法性,$sigma(n v) = n sigma(v), n in NN$
+ 进一步,将有:$sigma(-n v) + sigma(n v) = 0 => sigma(-n v) = -n sigma(v)$
+ 断言 $sigma(q/p v) = q/p sigma(v)$,事实上:
$
p sigma(q/p v) = sigma(p q/p v) = sigma(q v) = q sigma(v) => sigma(q/p v) = q/p sigma(v)
$
+ 这提示我们可以采用柯西方程,只需要额外的单调性或者连续性的条件即可
- 无论何者,证明的要点都是证明若 $sigma(lambda alpha) = u sigma(alpha)$,则对任意向量 $beta$,均有:
$
sigma(lambda beta) = u sigma(beta)
$
- 若 $alpha, beta$ 不共线,利用平行线分线段成比例易证
- 若共线,只需再作一个与其不共线的向量作为桥梁
- 有了上面的结论,证明单调性只需:
取 $sqrt(lambda) alpha -> u' sigma(alpha)$,即有 $lambda alpha = sqrt(lambda) sqrt(lambda) alpha -> u'^2 alpha => u >= 0$
- 利用保序性很容易证明单调性,只要对每个趋于零的向量列找到一个略大一点且像趋于零的向量列控制即可
+ 对一般的 $x in R$,我们可以找两列有理数:
$
q_1, q_2, ..., q_n,... -> x and < x\
p_1, p_2, ..., p_n,... -> x and > x
$
从而利用单调性条件:
$
|sigma(q_i v)| = |q_i| |sigma(v)| < |sigma(x v)|\
|sigma(p_i v)| = |p_i| |sigma(v)| > |sigma(x v)|
$
或者连续性条件:
$
|sigma(q_i v)| -> |sigma(x v)|
$
都可以得到结论
]
#corollary[][
变换 $sigma$ 保持分比当且仅当它是仿射变换
]
#proposition[][
$"Aff"(EE^n) -> "GL"(V(EE^n))$,也即仿射变换群到向量的线性变换群存在自然的满同态
]
#theorem[仿射变换的坐标表示][
设 $sigma: EE^3 -> EE^3$ 是仿射变换,$(O, e_1, e_2, e_3)$ 是一个仿射标架,则对任意点 $P: O + overline(O P)$,将有:
$
sigma(P) = sigma(O) + sigma(overline(O P))
$
从而若设 $overline(O P) = (e_1, e_2, e_3)X$,将有:
$
sigma(P) = sigma(O) + (sigma(e_1), sigma(e_2), sigma(e_3))X\
= O + (e_1, e_2, e_3)beta + (e_1, e_2, e_3)C X
$
换言之,$sigma(P)$ 的坐标 $X'$ 为:
$
X' = C X + beta
$
其中 $(e_1, e_2, e_3)C = (sigma(e_1), sigma(e_2), sigma(e_3))$
]
#corollary[][
任何平面上保持某个线向不变的仿射变换或者有不动点,或者有不变的直线
]
#proof[
- $X$ 是不动点当且仅当:
$
(I - C)X = beta
$
- $X_0 + t v$ 是不动直线当且仅当:
$
C X_0 + beta - X = t v <=>
(I - C)X_0 = beta - t v\
and (I - C)v = 0
$
设方程(1)无解,则 $"rank"(I - C) <= 1$,从而 $(I - C)v = 0$ 有解。
- 若 $"rank"(I - C) >= 1$,则由 $1$ 知 $beta in.not im(I-C)$,进而 $beta plus.circle im(I-C) = FF^2$
- 若 $"rank"(I - C) = 0$,则 $C = I$,进而是平移,显然有不变直线
]
设 $"Aff"(EE^n)$ 是仿射变换群:
- 保距变换 $"Isan"(EE^n)$ 是其子群
- 平移变换群 $"Trans"(EE^n)$ 是其交换的正规子群
- 一般而言,轴不同的旋转不可交换,因此保距变换群不交换。同时它也不是正规子群。
设空间所有图形构成集合 $X$,空间上的变换自然产生其上的一个群作用,某个稳定子群称为保持某图形不变的变换群
#example[][
求保持:
$
(sqrt(x^2 + y^2) - a)^2 + z^2 = r > 0
$
的仿射变换。
由于这是一个圆台,保持它的仿射变换应当当然保持圆台的对称轴不变。这因为任取两对同一平面上的对径点,它们的交点是各自的中点,而仿射变换保持共面,相交关系和中点不变,因此它们的像也是同一平面上的对径点,进而变换保持该平面的圆心不变。\
]
== 克莱因纲领
克莱因纲领是黎曼几何之前的古典几何的一种经典归纳。几何空间上的可逆变换构成一个群,不同的几何就是研究在某个子群下不变的性质。
换言之,这个在这个子群下,所有处于同一轨道的图形都认为是相同的图形。注意到处于同一轨道的图形可以通过变换相互到达,因此这个子群是图形的等价关系的等价类。\
同时,若 $H <= G$,则 $G$ 的轨道只会比 $H$ 更小。进而若两个图形在 $G$ 中等价,则在 $H$ 中也等价,同时若函数在 $G$ 中所有变换下不变,则当然在 $H$ 的所有变换下不变,
例如取 $G = "Aff"(EE^3), H = Isom(EE^3)$,就是说保距变换下等价的图形当然仿射等价,或者保距变换的不变量当然是仿射变换的不变量。\
#example[欧氏几何/度量几何][
欧氏几何就是研究保距变换下不变的性质,包括:
- 距离
- 角度
- 面积
- 其他仿射几何中的几何性质
笛卡尔坐标系是经典的欧氏几何模型,某个几何中不变的性质当然应该在不同的模型中都不变,因此可以利用坐标系来研究几何性质。
]
#example[球面几何][
在保距变换群中,取得一个保持单位球面不变的子群,在这个子群下不变的性质称为球面几何。
]
#example[仿射几何][
仿射几何就是研究仿射变换下不变的性质,包括:
- 平行
- 相交
- 简单比
- 交比
]
#example[射影几何][
后半学期会学习射影几何,它研究射影变换下不变的几何。
]
= 射影几何
== 中心投影
#definition[中心投影][
设 $pi_1, pi_2$ 是两个平面,$O$ 是平面外一点。任取 $pi_1$ 上一点 $X$,$O X$ 两点唯一决定一条直线 $L_X$,它交平面 $Pi_2$ 于点 $Y$。显然这大致构造了一个映射 $phi: pi_1 -> pi_2$ \
不幸的是,仅有若干条平行于 $pi_2$ 的直线 $L_X$ 无法确定 $Y$,因此我们必须暂时去掉这些点。
]
#proposition[][
- 中心投影保持直线
- 中心投影不保持直线的相交、平行
]
#proposition[][
选取圆锥的顶点做中心投影,圆锥上所有二次曲线都可以由不同平面的中心投影得到,进而中心投影保持二次曲线
]
== 射影平面
#definition[扩大的欧氏平面/线向完备化模型][
在中心投影中,在 $pi_2$ 上人为补充所有经过点 $O$,平行于 $pi_2$ 的直线作为无穷远点,这些无穷远点和通常点合在一起构成了一个扩大的平面,称为扩大的欧氏平面,这样的平面统称射影平面,这个构造称为线向完备化模型。
]
在射影平面之间,中心投影可以给出完备的几何变换。这种变换保持点在线上,线过点等性质。注意这种变换不需要保持平行,这可以为我们证明某些只与点在线上,线过点的定理提供极大的便利。
#proposition[射影平面的基本性质][
+ 任何两点确定一条直线
+ 任何两条直线确定一个点
+ 中心投影是两射影平面间的一一映射,并将直线映为直线
]
#definition[射影平面的线把模型][
设 $O$ 是空间上任意一点,$pi$ 是不过 $O$ 的某个投影平面,其上的点与过 $O$ 点的所有直线有一一对应关系,称为射影平面的线把模型。(事实上,这与平面的选取无关,选取不同平面相当于做了一个投影变换)
]
#definition[射影平面的齐次坐标][
线把模型中,显然每个直线可以记作一个三维向量 $vec(a, b, c)$。实际上,它的所有取值相当于:
$
quotient(RR^3 - {0}, (alpha ~ RR alpha))
$
往往将其中元素记作 $HomoCoor(x, y, z) = RR vec(x, y, z)$,这被称为射影平面的齐次坐标。
]
#remark[][
可以看出我们也可以只取单位向量,这就与以 $O$ 为球心的单位球面上的点构成对应(一对对径点恰对应一条直线)。在这个模型下,点就是球面上一对对劲点,平面就是过球心的平面或者一个大圆面。
]
#remark[][
射影空间是性质很好的空间,例如在代数几何中,射影空间可以完全被内蕴地给出代数定义,但常见的仿射几何则不行。
]
== 射影坐标系
#remark[][
这节及以后讨论的所有齐次坐标都要求非零
]
在线把模型中,每条过 $O$ 的直线唯一确定一个齐次坐标,同时也唯一确定一个射影平面上的点,因此射影平面上的点与齐次三维向量产生一一对应,这被称为射影平面的齐次坐标。
#proposition[][
-
$HomoCoor(x, y ,z), autoHomoCoor3(a), autoHomoCoor3(b)$ 共线
\
$<=> HomoCoor(x, y ,z) = lambda autoHomoCoor3(a) + mu autoHomoCoor3(b), lambda, mu != 0$\
$<=> mat(delim:"|",
x, a_1, b_1;y, a_2, b_2;z, a_3, b_3) = 0$\
$<=> v^T HomoCoor(x, y ,z) = 0$,其中 $v$ 由行列式展开给出
]
#remark[][
从线把模型的角度,一条直线对应一个平面,而过原点的平面坐标恰如上式。
]
#proposition[][
在扩大的仿射平面模型中,可以将仿射平面的坐标嵌入射影平面坐标:
$
vec(x, y) <-> HomoCoor(x, y, 1)
$
同时,无穷远直线恰为:
$
[0, 0, 1]X = 0
$
]
#proposition[射影标架][
对于射影平面上任意一般位置四点 $A, B, C, D$,存在唯一射影标架使得四点坐标分别为:
$
HomoCoor(1, 0 ,0), HomoCoor(0, 1 ,0), HomoCoor(0, 0 ,1), HomoCoor(1, 1 ,1)
$
称此标架为由四点构成的射影标架
]
#proof[
仍然考虑线把模型,设 $A, B, C, D$ 分别由空间向量 $v_1, v_2, v_3, v_4$ 确定,有:
$
v_4 = lambda_1 v_1 + lambda_2 v_2 + lambda_3 v_3
$
由一般位置知这些参数非零
则对射影平面上任意一点 $P$,对应向量 $v$,将有:
$
v = (1/lambda_1 v_1, 1/lambda_2 v_2, 1/lambda_3 v_3)X
$
从而对应一个射影标架:
$
P = [1/lambda_1 v_1, 1/lambda_2 v_2, 1/lambda_3 v_3] X
$
容易看出在这个标架下,满足 $A, B, C, D$ 四点坐标恰为:
$
HomoCoor(1, 0 ,0), HomoCoor(0, 1 ,0), HomoCoor(0, 0 ,1), HomoCoor(1, 1 ,1)
$
因此满足要求,\
对于唯一性,设有一空间仿射标架为:
$
v = (alpha_1, alpha_2, alpha_3 )X
$
由于:
$
(v_1, v_2, v_3) = (alpha_1, alpha_2, alpha_3 ) mat(k_1, 0, 0;0, k_2, 0;0, 0, k_3)
$
由于 $v_1, v_2, v_3$ 线性无关,因此矩阵可逆,故可设(显然 $k_i !=0$):
$
alpha_i = 1/k_i v_i
$
同时:
$
v_4 = k (alpha_1, alpha_2, alpha_3)vec(1, 1, 1)\
= (k/k_1 v_1, k/k_2 v_2, k/k_3 v_3)vec(1, 1, 1)
$
而 $v_4$ 被 $v_1, v_2, v_3$ 表出方式是唯一的,这意味着:
$
(k/k_1 v_1, k/k_2 v_2, k/k_3 v_3) = mu (1/lambda_1 v_1, 1/lambda_2 v_2, 1/lambda_3 v_3) \
[alpha_1, alpha_2, alpha_3] = [1/lambda_1 v_1, 1/lambda_2 v_2, 1/lambda_3 v_3]
$
这就证明了唯一性
]
== 射影映射
#definition[射影映射][
在两个射影平面上,将直线映为直线的映射的可逆映射称为射影映射,同一个平面上的射影映射称为射影变换。
]
#example[][
- 仿射映射是射影映射,它比射影映射的定义恰好多了保持直线平行
- 中心投影是射影映射
]
#theorem[][
射影映射的逆映射还是射影映射
]
#proof[
设 $P$ 设射影映射,$A', B', C'$ 是直线 $l'$ 上不同三点,且 $P(A, B, C) = (A', B', C')$\
若 $A, B, C$ 三点不共线,则至少一个不是无穷远点,不妨设为 $A$。\
记直线 $A C$ 为 $Sigma_1$,直线 $B C$ 为 $Sigma_2$,显然有:
$
P(Sigma_1) subset l'\
P(Sigma_2) subset l'
$
再任取 $D in Sigma_1, E in Sigma_2$,则有:
$
P(D E) subset l'
$
这将意味着过两不重合直线 $Sigma_1, Sigma_2$ 上任意两点确定的直线的像都在 $l'$ 上,这是荒谬的。
]
#corollary[][
射影映射 $P$ 不会将两条不同直线映到同一直线上,进而射影映射诱导出射影平面上直线之间的一一映射。
]
#corollary[][
空间中保持一点不动并将平面映成平面的可逆映射诱导出平面间的一一对应关系
]
#remark[][
- 设 $sigma$ 是平面 $Sigma$ 上仿射变换,$Sigma'$ 是扩大的欧氏平面,则令:
$
P(X) = cases(
sigma(X) space X in Sigma,
X space X in Sigma' - Sigma
)
$
也就是补充将无穷远点映为无穷远点,这将成为 $Sigma'$ 上的射影映射,并将无穷远直线映为无穷远直线。
- 反之,设 $P$ 是 $Sigma'$ 上将无穷远直线映为无穷远直线的射影映射,则 $P|_Sigma$ 将成为在欧氏平面上保持直线的映射,进而它是仿射映射。
- 进一步,设 $Sigma'$ 是扩大的欧氏平面,则对任意一条直线 $l$,存在射影变换 $P$,它把无穷远直线映为 $l$。只需要取中心投影将无穷远直线变为有限直线,再取仿射映射变为指定直线即可。这表明在射影映射下,无穷远直线与通常直线实际上没有什么区别。
- 事实上,任意射影平面去掉一条直线就变成了仿射平面,在这个仿射平面中不相交的直线称为仿射平面中的平行直线。显然这个平行关系与具体去掉哪个直线有关,因此需要在上下文无歧义时使用。
]
若将仿射变换群 $Hom(pi)$ 视作射影变换群 $Proj(pi)$ 的子群,则有:
$
Proj(pi) = union.big_(i) sigma_i Hom(pi)
$
其中 $sigma_i$ 是将某条通常直线映为无穷远直线的射影变换。
#proposition[][
取 $sigma$ 是线把模型中射影平面上的点与过 $O$ 点的直线的一一对应,$phi$ 是 $EE^3$ 中仿射变换,将有:
$
phi' = Inv(sigma) dot phi dot sigma
$
给出射影平面上一个射影变换。\
] <proj1>
#proof[
首先 $sigma, phi$ 都是双射,故 $phi'$ 也是双射。\
而:
$phi'$ 是射影变换 $<=> phi'$ 保持射影平面上直线\
$<=> phi dot sigma$ 将射影平面上直线映成空间中平面\
$<=>$ $phi$ 将空间中平面映成平面
最后一句由仿射映射的性质是显然的。
]
#proposition[][
命题的反面也成立,也即任意给定射影平面上射影变换 $P$,存在保持 $O$ 点不动的映射 $phi$ 使得 $P = Inv(sigma) dot phi dot sigma$ 且 $phi$ 为仿射映射
] <proj2>
#proof[
#lemma[][
空间中将零向量映成零向量,并保持向量共面关系的可逆向量变换一定是线性变换
]
#proof[
首先,从之前射影变换的逆变换还是射影变换可以看出,这样的变换的逆变换还保持向量共面关系不动。
只需证明这样的变换保持向量共线,进而由仿射变换的熟知结论知它是线性变换。\
记满足要求的向量映射为 $f$,用反证法,假设 $a, b$ 共线但 $f(a), f(b)$ 不共线,取 $f(c) = f(a) times f(b)$,则 $f(a), f(b), f(c) "不共面" <=> a, b, c "不共面"$,但由 $a, b$ 共线,这是荒谬的。
另证:对任意向量 $alpha$,找 $beta, gamma$ 使得 $alpha, beta, gamma$ 线性无关,从而:
$
dim(generatedBy(alpha\, beta)) = dim(generatedBy(alpha\, gamma)) = 2
$
设 $V_1 = generatedBy(alpha\, beta), V_2 = generatedBy(alpha\, gamma)$,将有(注意到 $f$ 可逆):
$
V_1 sect V_2 = generatedBy(alpha)\
f(V_1 sect V_2) = f(V_1) sect f(V_2)
$
($f(V_1), f(V_2)$ 仍是线性空间,这是因为 $V_1$ 是所有与 $alpha\, beta$ 共面的向量,经过映射仍然共面。同时考虑逆映射,所有与 $f(alpha), f(beta)$ 共面的向量的原像也与 $alpha\, beta$ 共面)
- $V_1 != V_2 => f(V_1) != f(V_2)$,故 $dim(f(V_1) sect f(V_2)) < 2$
- $f(alpha) in f(V_1) sect f(V_2), f(alpha) != 0 => dim(f(V_1) sect f(V_2)) >= 1$
这表明 $dim(f(V_1) sect f(V_2)) = 1$,进而 $f(V_1 sect V_2) = f(V_1) sect f(V_2) = f(generatedBy(alpha))$\
这就证明了 $f$ 保持向量共线。\
(另证中的方法容易推广至一般空间,但本质是相同的)
]
考虑以 $O$ 为原点的所有向量,引理表明将过 $O$ 点平面映射成过 $O$ 点平面的空间变换一定是保持 $O$ 点不动的仿射变换。但我们之前使用的 $sigma$ 表示直线间的映射,这里我们必须明确给出一个点变换(或者向量变换),下面的命题将详细给出定义:
#proposition[][
给定 $EE^3$ 上保持共面关系的所有过 $O$ 直线间的一一对应 $phi$,则存在可逆的向量变换 $f$,使得:
$
phi(l_(A O)) = l_(f(A) O) <=> f(l) = phi(l)
$
同时,这给出 $f$ 保持向量共面关系。
构造是容易的,只需考虑上半球面挖去底面的右半部分构成的集合 $S$,它与过 $O$ 点所有直线恰交于一点。记 $S_l$ 为 $S$ 与直线 $l$ 的交点,定义向量变换 $f$:
$
f(lambda overline(O S_l)) := lambda overline(O S_(phi(l)))
$
由于空间中所有向量都可以唯一写作 $lambda overline(O S_l)$,因此 $f$ 是良定义的。并且容易验证,$Inv(phi)$ 诱导出的向量映射恰为 $Inv(f)$(注意之前我们已经论述,空间中保持平面关系的直线变换的逆仍然保持平面关系)\
同时保持共面关系是显然的,因为:
$
lambda_1 overline(O S_l_1), lambda_2 overline(O S_l_2), lambda_3 overline(O S_l_3) "共面"\
<=> l_1, l_2, l_3 "共面" \
<=> phi(l_1), phi(l_2), phi(l_3) "共面"\
<=> lambda_1 overline(O S_(phi(l_1))), lambda_2 overline(O S_(phi(l_2))), lambda_3 overline(O S_(phi(l_3))) "共面"\
<=> f(lambda_1 overline(O S_l_1)), f(lambda_2 overline(O S_l_2)), f(lambda_3 overline(O S_l_3)) "共面"
$
]
这与我们最终要证明的命题已经很接近了,取 $sigma$ 是射影平面上点与过 $O$ 点直线一一对应,由于 $P$ 是射影变换,因此:
$
sigma dot P dot Inv(sigma)
$
保持直线共面关系,进而由上述命题诱导出向量变换 $f$ 且保持向量共面关系。由引理,$f$ 就是保持 $O$ 点不动的仿射变换。\
另一方面,由命题:
$
(Inv(sigma) dot f dot sigma)(l) = Inv(sigma) dot f(sigma(l)) = Inv(sigma) dot (sigma dot P dot Inv(sigma))(sigma(l)) = P(l)
$
最终证明了原结论。
]
上面两个命题结合,我们得到了最重要的定理:
#theorem[][
设 $sigma$ 是线把模型中射影平面上点与过 $O$ 点直线一一对应,则保持 $O$ 点不动的仿射变换群 $Hom(O)$ 与射影平面上射影变换群 $Proj(pi)$ 有满同态 $pi.alt$,并且满足:
$
f(sigma(X)) = sigma(pi.alt(f)(X))
$
同时,$ker(pi.alt)$ 恰为所有以 $O$ 为中心的伸缩映射,进而由第一同构定理:
$
quotient(GL_3, RR I) tilde.eq quotient(Hom(O), ker(pi.alt)) tilde.eq Proj(pi)
$
]
#proof[
根据 @proj1,只需取 $pi.alt(f) = Inv(sigma) dot f dot sigma$ \
由于 $(Inv(sigma) dot f dot sigma) dot (Inv(sigma) dot g dot sigma) = Inv(sigma) dot (f dot g) dot sigma$,因此这确实是群同态,而条件:
$
f(sigma(X)) = sigma(pi.alt(f)(X))
$
是显然成立的。\
同时 @proj2 表明这是满射,因此是满同态。\
显然,$ker(pi.alt)$ 满足空间中所有向量都是特征向量,这表明它就是所有以 $O$ 为中心的伸缩映射,矩阵表示恰为 $RR I$
]
#corollary[射影变换的矩阵表示][
在上面的定理中,$sigma(X)$ 实际上就是 $X$ 的坐标表示 $autoHomoCoor3(x)$,因此射影映射 $P$ 可以被表示为:
$
P(autoHomoCoor3(x)) = A autoHomoCoor3(x)
$
其中 $A$ 是 $Inv(pi.alt)(P)$ 中某个元素的矩阵表示,进而在相差倍数的意义下唯一。
]
#theorem[][
- 射影映射由四个处于一般位置的点唯一确定。
- 射影变换一定可以写作若干中心投影的复合
]
#proof[
设四点 $A, B, C, D$ 的像为 $A', B', C', D'$。
#lemma[][
空间内不同射影平面间的平移,旋转可以用中心投影实现
]
#proof[
两者的操作类似,由于变换前后的图形对称,取其对称面。先投影一次投影到对称面,再投影一次到新平面,容易验证结果与平移/旋转是相同的。
]
#lemma[][
可以通过中心投影使得 $A, B, C, D, A', B', C', D'$ 都是通常点
]
#proof[
只需要考虑无穷远直线及它的像。容易找到两条直线,$A, B, C, D;A', B', C', D'$ 分别不在其上,可以通过中心投影保证这两条直线恰为无穷远直线,此时这些点当然不是无穷远点。
]
由引理,可设这些点都是处于一般位置的通常点。我们依次确定四点的像:
- 首先,容易做中心投影将 $A$ 映成 $A'$
- 其次,任找一条过 $A'$ 且不过其他点的直线,绕这个直线对平面任做旋转,再任做中心投影都保持 $A'$ 不动,容易将 $B$ 的像调整到 $B'$
- 类似的,可以绕 $A' B'$ 旋转,将 $C$ 的像调整到 $C'$
- 至此,存在射影变换保持 $A B C$ 三点,并将 $D$ 映成 $D'$
运用代数方法,设:
$
overline(O D) = lambda_1 overline(O A) + lambda_2 overline(O B) + lambda_3 overline(O C)\
overline(O D') = mu_1 overline(O A) + mu_2 overline(O B) + mu_3 overline(O C)
$
注意到由于四点处于一般位置,这些系数都非零\
则考虑空间仿射变换:
$
overline(O A) -> mu_1/lambda_1 overline(O A)\
overline(O B) -> mu_2/lambda_2 overline(O B)\
overline(O C) -> mu_3/lambda_3 overline(O C)
$
它诱导的射影变换将保持 $A B C$ 三点,并将 $D$ 映成 $D'$,这就证明了第一个结论。\
注意到这个仿射变换相当于将平面 $A B C$ 中心投影到平面 $A' B' C'$,其中:
$
overline(O A') = lambda_1/mu_1 overline(O A)\
overline(O B') = lambda_2/mu_2 overline(O B)\
overline(O C') = lambda_3/mu_3 overline(O C)
$
此时将有:
$
overline(O D) = mu_1 overline(O A') + mu_2 overline(O B') + mu_3 overline(O C')\
$
进而 $D$ 在该平面上投影相对 $A', B', C'$ 将恰为 $D'$,将该平面通过平移,旋转(由中心投影实现)变成原平面,这就证明了第二个结论。
对于唯一性,注意到:
$
A autoMat3(x, y,z, w) = autoMat3(a, b,c,d)mat(k_1, 0, 0, 0;0, k_2, 0, 0; 0, 0, k_3,0;0, 0, 0, k_4)
$
设 $w = lambda_1 x + lambda_2 y + lambda_3 z$,(由于四点处于一般位置 $lambda_i != 0$)则对两侧同时列变换得:
$
A mat(a_1, b_1, c_1, 0;a_2, b_2, c_2, 0;a_3, b_3, c_3, 0) = autoMat3(a, b,c,d)mat(k_1, 0, 0, -k_1 lambda_1;0, k_2, 0, -k_2 lambda_2; 0, 0, k_3,-k_3 lambda_3;0, 0, 0, k_4)
$
当且仅当 $k_4 d = k_1 lambda_1 a + k_2 lambda_2 b + k_3 lambda_3 c$ 时有解。由于 $a, b, c$ 是一组基,因此这样的 $k_i$ 在差一系数的意义下唯一,进而射影变换唯一。
]
#remark[][
所有的仿射变换都(延拓意义下)是射影变换,从而在射影意义上可以由中心投影实现
]
#remark[][
- 在欧氏几何中,有等腰三角形,一般三角形;平行四边形,菱形,正方形,凸四边形等等概念,它们在等距变换下保持不变
- 在仿射几何中,所有的三角形共属一类,四边形还可区分(所有平行四边形共属一类,凸四边形概念依然存在)
- 在射影几何中,所有的四边形共属一类。
]
#remark[][
我们所有对射影映射(变换),包括之前对仿射映射的讨论 *都基于由实数实现的线性空间*。有些性质是无法推广的,例如在复数域存在非平凡同构,这导致保持向量共线的映射未必成为线性映射,进而保持直线的映射未必有矩阵表示,射影映射也会有同样的问题,有关定义的具体含义可能会发生变化。
]
== 对偶原理
设 $Sigma$ 是射影平面,$Sigma^*$ 是所有 $Sigma$ 上的直线构成的集合,称为 $Sigma$ 的对偶平面。*对偶原理实际上是说射影空间与其对偶空间(在线性关系上)是同构的*。\
利用线把模型,定义如下映射 $phi: Sigma -> Sigma^*$,它将射影点 $X$(对应过中心直线 $l$)映为射影直线 $l$(对应过 $X$ 的平面,这个平面恰与 $l$ 垂直)。\
容易验证这样定义的映射 $phi$ 是一一的,且满足:
$
X, Y, Z "三点共线" <=> phi(X), phi(Y), phi(Z) "三线共点"
$
换言之,$Sigma^*$ 也可以看作射影平面,它的几何性质与 $Sigma$ 是相同的。这也意味着,射影平面上任何关于点、线的命题都有对偶的线、点的命题,且它们同时为真/假,这就是对偶原理。
#example[迪沙格定理][
- 两三角形对应顶点的连线共点 $=>$ 两三角形对应边的交点共线
- 两三角形对应边的交点共线 $=>$ 两三角形对应顶点的连线共点
这两个事实是互相对偶的,它们同时为真。
]
#remark[][
对偶原理只能作用于纯线性的命题,不能直接应用于二次相关的命题。
]
== 分比
分比是射影变换重要的不变量,同时也是本质的不变量。\
回顾 @简单比 简单比,它是仿射变换的不变量,但是在射影几何下不是。考察平面投影,设 $A, B, C$ 投影为 $A', B', C'$ 可以发现:
$
((A, B, C))/((A', B', C'))
$
是与 $C$ 点无关的。因此再取一点 $D$ 便有:
$
((A, B, C))/((A', B', C')) = ((A, B, D))/((A', B', D'))\
<=> ((A, B, C))/((A, B, D)) = ((A', B', C'))/((A', B', D'))
$
表明后者是一个射影不变量,称其为分比
#definition[仿射平面上的分比][
- 设 $A, B, C, D$ 四点共线,且不允许任意三点相同,则记:
$
(A, B;C, D) = ((A, B, C))/((A, B, D)) = (arrow(A C)/arrow(C B))/(arrow(A D)/arrow(D B))
$
为四点的分比
- 设 $l_1, l_2, l_3, l_4$ 共点于 $O$,且不允许任意两点相同,任取一条之间 $l$ 截四线于 $A, B, C, D$,由上面的论述这与所选 $l$ 无关,因此记:
$
(l_1, l_2; l_3, l_4) = (A, B;C, D)
$
为四线的分比
- 设 $v_1, v_2, v_3, v_4$ 是向量,且:
$
v_3 = s_1 v_1 + t_1 v_2\
v_4 = s_2 v_1 + t_2 v_2\
$
则定义它们的分比为:
$
(v_1, v_2;v_3, v_4) := (t_1 / s_1)\/(t_2 / s_2)
$
它与上面两个定义是相容的
]
#proposition[欧式平面上直线分比的角度度量][
取逆时针为正,设 $l_1, l_2, l_3, l_4$ 之间的夹角依次为 $theta_(i j)$,则有:
$
(l_1, l_2; l_3, l_4) = ((sin theta_(1 3))/(sin theta_(3 2)))/((sin theta_(1 4))/(sin theta_(4 2)))
$
]
#proof[
由于选取直线不影响分比,因此选取直线 $l$ 使得 $|O A| = |O B|$。 使用正弦定理计算面积易得:
$
(A, B, C) = (sin theta_(1 3))/(sin theta_(3 2))\
(A, B, D) = (sin theta_(1 4))/(sin theta_(4 2))
$
从而结论成立
]
#theorem[][
分比有以下运算性质:
- $1/((A_1, A_2;A_3, A_4)) = (A_2, A_1;A_3, A_4) = (A_1, A_2;A_4, A_3)$
- $1- (A_1, A_2;A_3, A_4) = (A_1, A_3;A_2, A_4)$
- $(A_1, A_2;A_3, A_4) = (A_3, A_4;A_1, A_2)$
]
#definition[扩大的欧式平面上的分比][
对共线四点 $A, B, C, D$
- 若它们都是通常点,按照仿射平面情形定义分比
- 若只有 $D$ 是无穷远点,定义:
$
(A, B;C, D) = (A, B, C)
$
- 若它们都是无穷远点,则取四个点对应无穷远方向的共点直线,定义四点交比是四个直线的交比
对于共点四线 $l_1, l_2, l_3, l_4$:
- 若它们都是通常直线,则按仿射平面情形定义
- 若 $l_4$ 是无穷远直线,此时另外三条直线平行,定义分比是任取一条直线截三条平行直线所得交点的简单比(显然与所选直线无关)
]
#definition[线把模型中的分比][
设 $A, B, C, D$ 是共线四点,定义:
$
(A, B;C, D) = (O A, O B; O C, O D)
$
右侧是扩大的射影平面上四线的交比,且与 $O$ 点的选取无关
对于共点四线,定义分比为任取一条直线截四线所得点的分比
]
#definition[调和点列/线束][
设 $A, B, C, D$ 是共线四点,若:
$
(A, B;C, D) = - 1
$
则称四点成为调和点列,同时称 $D$ 是 $C$ 关于 $A, B$ 的调和共轭点。类似定义调和线束。
]
#theorem[][
交比是射影不变量。也即设 $A, B, C, D$ 在射影映射 $phi$ 下的像为 $A', B', C', D'$,则:
$
(A, B;C, D) = (A', B';C', D')
$
]
#proof[
证明的方法非常多,例如从代数出发事实是容易的。这里从几何出发,先证明中心投影保持交比不变,再有射影映射是中心投影的复合知结论成立。
事实上,注意到线把模型中,射影平面上的射影变换就是空间中的仿射变换,它当然保持共点四线的交比,进而不改变仿射平面上四点的交比。
]
#remark[][
*以上所有定义都是相容的*,本质可以归结于向量的分比。同时,点的分比与线的分比的统一性也是符合对偶原理的。分比的概念其实相当古老,早在古希腊时期人们就知道了分比是中心投影的不变量,可以用来解决很多问题。
]
== 射影平面上的二次曲线
容易看出,射影平面上的二次曲线方程可以写作:
$
[x, y, z]A HomoCoor(x, y, z) = 0 := X^T A X = 0
$
#remark[][
射影平面中并不是所有的方程都确定一条曲线,容易看出良定义性当且仅当方程齐次。
]
在坐标变换:
$
HomoCoor(x, y, z) = T HomoCoor(x', y', z')
$
下,方程变为:
$
[x', y', z']T^T A T HomoCoor(x', y', z')
$
由于 $T$ 可以任取可逆矩阵,因此射影平面上二次曲线的等价类就是合同变换下的等价类(允许差一个非零常数,注意这也意味着正负号不做区分)。
而代数上可以证明,矩阵的合同等价类唯一由正惯性指数和负惯性指数确定,因此枚举所有正负惯性指数 $(p, q)$:
- $(3, 0) ~ (0,3): x^2 + y^2 + z^2 = 0$:这是空集(注意射影坐标中三点不同时为零)
- $(1,2) ~ (2, 1): x^2 + y^2 - z^2 = 0$:蕴含了所有仿射几何中的通常二次曲线,包括椭圆,双曲线,抛物线。常常也使用另一种标准型 $x z - y^2 = 0$
- $(0, 2) ~ (2, 0): x^2 + y^2 = 0$:空集
- $(1, 1): x^2 - y^2 = 0$:这是一对相交直线
- $(1, 0) ~ (0,1): x^2 = 0$:这是二重直线
#remark[][
- 这表明实数域下,所有(非退化的)圆锥曲线都是射影等价的,这个事实是可以用几何方法另证:
设 $Sigma$ 是空间中一个圆锥曲线,显然它是圆锥与某个平面的交线。取中心投影容易将其变为椭圆。而所有的椭圆都仿射等价,当然也是射影等价的。
- 在复数域下,所有满秩矩阵都合同于单位矩阵,因此分类结果可以简化为三种。
]
二次曲线究竟由多少个点确定是我们所关心的问题,有许多方法可以得到相同的结论。
#proposition[二次曲线是有理曲线][
非退化二次曲线是可以参数化的。更精确地说,对于任何非退化二次曲线 $Tau$,存在某个坐标使得 $Tau$ 恰为:
$
{x z - y^2 = 0} <=> {HomoCoor(u^2, u v, v^2) | (u, v) in RR^2}
$
并且在差一个非零常数的意义下参数是唯一的。\
在通常的仿射几何中,往往也可以找到一个有理分式对二次曲线进行参数化。
]
#theorem[][
+ 两个非退化二次曲线至多有四个交点。若在复数域上且考虑重数,则恰有四个交点
+ 若一个至少包含一个点的二次曲线的两个方程分别为 $F_1 = 0, F_2 = 0$,则 $F_1 = c F_2$
+ 平面上处于一般位置的五个点唯一确定一条二次曲线
+ 设 $A_1, A_2$ 是两个非退化二次曲线,有四个交点(考虑重数),则所有过这四个交点的二次曲线即为:
$
X^T (lambda A_1 + mu A_2) X = 0
$
]
#proof[
带有重数的命题比较复杂,这里均不证明,只证明四个交点不同的情况。
- (1)从参数化方程可以看出,两个二次曲线联立将得到四次(齐次)方程,显然最多有四组解。
- (2)由(1)易得
- (3)唯一性由(1)是易得的,存在性考虑关于 $A$ 的矩阵方程:
$
V_i^T A V_i = 0
$
这是含有 $6$ 个变量,$5$ 个方程的线性方程组,它当然有解。
- (4)设四个交点不同,仿照上面构造线性方程组,过四个点的二次曲线解空间维度为 $2$,恰好就是 $generatedBy(A_1\, A_2)$
]
#corollary[][
平面上不在同一直线上的五点唯一确定一个圆锥曲线
]
从更加线性代数的角度也可以给出证明,同时将退化情形容纳进去
#lemma[][
设 $X_1, X_2, X_3, X_4$ 是处于一般位置四点,则:
$
"rank" (mat(x_1^2, y_1^2, z_1^2, x_1 y_1, y_1 z_1, x_1 z_1;
x_2^2, y_2^2, z_2^2, x_2 y_2, y_2 z_2, x_2 z_2;
x_3^2, y_3^2, z_3^2, x_3 y_3, y_3 z_3, x_3 z_3;
x_4^2, y_4^2, z_4^2, x_4 y_4, y_4 z_4, x_4 z_4;)) = 4
$
]
#proof[
可以验证这个矩阵的秩在对四点做同一个可逆线性变换的情况下不变,进而不妨假设四点是最简单情形 $vec(1, 0 ,0), vec(0, 1 ,0),vec(0, 0 ,1),vec(1, 1 ,1)$,结论显然成立
]
这立刻表明所有过一般位置四点的二次曲线构成二维线性空间,自然上面的结论都成立\
从几何角度证明需要用到如下的定理
#theorem[Steiner][
设 $A_1, A_2, A_3, A_4$ 是射影平面上圆锥曲线上任意四个不同点,任取 $P$ 在圆锥曲线上,则:
$
(P A_1,P A_2; P A_3, P A_4)
$
恰为一个定值(规定若 $P = A_i$,则 $P A_i$ 是该点处的切线),且符合交比等于此定值的所有点恰为圆锥曲线上所有点。
]
#proof[
用设射影变换,将圆锥曲线变为正圆。在正圆的情形用角度表示交比,由圆周角相等,结论是非常容易的。另一方面需要利用同一法证明。\
]
#remark[][
假设使用之前代数方法的结论,可以给出一个代数的证明:\
设 $B A_i^T = B times A_i$,且:
$
B A_3 = lambda_1 B A_1 + lambda_2 B A_2\
B A_4 = mu_1 B A_1 + mu_2 B A_2
$
则:
$
vec(B A_3^T, B A_4^T) = mat(lambda_1, lambda_2; mu_1, mu_2) vec(B A_1^T, B A_2^T)\
vec(B A_3^T, B A_4^T)(A_1, A_2, A_3, A_4) = mat(lambda_1, lambda_2; mu_1, mu_2) vec(B A_1^T, B A_2^T)(A_1, A_2, A_3, A_4) \
mat([B, A_3, A_1], [B, A_3, A_2],0, [B, A_3, A_4];[B, A_4, A_1], [B, A_4, A_2], [B, A_4, A_3], 0) = mat(lambda_1, lambda_2; mu_1, mu_2) mat(0, [B, A_1, A_2], [B, A_1, A_3], [B, A_1, A_4];[B, A_2, A_1], 0, [B, A_2, A_3], [B, A_2, A_4]) \
mat([B, A_3, A_1], [B, A_3, A_2];[B, A_4, A_1], [B, A_4, A_2]) = mat(lambda_1, lambda_2; mu_1, mu_2) mat(0, [B, A_1, A_2];[B, A_2, A_1], 0) \
mat(lambda_1, lambda_2; mu_1, mu_2) ~mat([B, A_3, A_1], [B, A_3, A_2];[B, A_4, A_1], [B, A_4, A_2]) mat(0, 1; -1, 0) = mat([B, A_2, A_3], [B, A_3, A_1];[B, A_2, A_4], [B, A_4, A_1])
$
因此分比恰为:
$
[B, A_1, A_3][B, A_2, A_4] \/ [B, A_1, A_4][B, A_2, A_3]
$
然而注意到
$
[B, A_1, A_3][B, A_2, A_4] = B^T (A_1 times A_3) (A_2 times A_4)^T B \
~ B^T ((A_1 times A_3) (A_2 times A_4)^T + (A_2 times A_4) (A_1 times A_3)^T) B
$
是过 $A_1, A_2, A_3, A_4$ 的二次曲线,分母同样处理,进而由之前结论:
$
[X, A_1, A_3][X, A_2, A_4] \/ [X, A_1, A_4][X, A_2, A_3] = k
$
对于不同的 $k$ 将唯一确定一个过 $A_1, A_2, A_3, A_4$ 的二次曲线,这就证明了结论。
]
#theorem[Pascal][
圆锥曲线上任意内接六边形的三组对边交点共线
]
#proof[
记 $A B C D E F$ 是一个内接六边形,三组对边分别为:
$
A B, D E := P\
B C, E F := Q\
C D, F A := R|
$
]
#proposition[][
设 $P$ 是圆锥曲线 $Sigma$ 的内部点,则存在射影变换 $phi$,使得:
- $phi(Sigma)$ 是圆
- $phi(P)$ 是 $phi(sigma)$ 的圆心
]
#proof[
考虑扩大的仿射平面,不妨设:
$
P = HomoCoor(0, 0, 1)
$
此时圆锥曲线方程为:
$
(x^T, z)mat(A, beta;beta^T, D)vec(x, z) = 0
$
我们的目标是找保持原点的射影变换 $H$,也就是形如:
$
mat(C, 0;alpha^T, e)
$<equ171>
的矩阵,将二次曲线变为标准的圆 $x^2 + y^2 - z^2 = 0$。\
不妨设圆锥曲线方程在仿射平面表示椭圆,也即存在可逆矩阵 $C$:
$
C^T A C = I
$
此时,@equ171 的形式恰好与仿射平面上非齐次坐标下的仿射变换统一,而通过这样的变换容易将任何一个椭圆变成标准的圆,这就证明了结论。
]
#definition[调和共轭][
对于不在圆锥曲线上的一点 $P$,过 $P$ 做直线 $l$ 交圆锥曲线于点 $A, B$,则直线上满足:
$
(P, Q;A, B) = -1
$
的点 $Q$ 称为点 $P$ 的调和共轭点,此时 $P$ 也是 $Q$ 的调和共轭点
]
#proposition[][
不在圆锥曲线上的一点 $P$ 的所有调和共轭点恰构成一条直线,称为 $P$ 的极线。若 $P$ 在圆锥曲线外部,则这条线恰好是两个切点的连线。特殊的,称圆锥曲线上一点的极线为它的切线。
]
#proof[
设圆锥曲线方程为 $X^T A X = 0$,$P$ 点坐标为 $X_0$,$Q$ 点坐标为 $X_1$,$l$ 方程为 $lambda X_0 + mu X_1$。\
联立:
$
(lambda X_0 + mu X_1)^T A (lambda X_0 + mu X_1) = 0 <=> lambda^2 X_0^T A X_0 + 2 lambda mu X_0^T A X_1 + mu^2 X_1^T A X_1 = 0
$<equ1>
先假设直线与圆锥曲线不相切,此时上述方程的两组解 $lambda_1, mu_1; lambda_2, mu_2$ 满足 $lambda_i != 0$\
因此注意到(利用韦达定理):
$
(P, Q;A, B) = (mu_1 / lambda_1) / (mu_2 / lambda_2) = -1 <=> mu_1 / lambda_1 + mu_2 / lambda_2 = 0 <=> (2 X_0^T A X_1)/ (X_1^T A X_1) = 0\
<=> X_0^T A X_1 = 0
$
对于给定的 $X_0$,所有满足 $X_0^T A X_1 = 0$ 的 $X_1$ 当然构成一条直线,这就证明了命题成立,同时也给出了极线的具体坐标公式。
对于相切的情形,只需注意到在 @equ1 中,$Q$ 是切点当且仅当 $Q$ 在圆锥曲线上,且:
$
X_0^T A X_1 = 0
$
这和刚刚的极线是同一条直线。同时若固定 $X_1$,则直线:
$
X_1^T A X = 0
$
恰好就是过 $Q$ 的切线
]
#proposition[][
圆锥曲线的焦点的极线就是准线
]
#definition[配极映射][
给定圆锥曲线 $sigma$,构造一一映射将点映为点的极线,它给出了射影平面与对偶平面的一个同构。
]
= Mobius 变换
== 反演
#definition[反演][
在 $EE^2$ 中,称以 $O$ 为圆心,$r$ 为半径的圆 $C$ 关于圆 $C$ 的反演为:\
$phi: EE^2 - {0} -> EE^2 - {0}$
它将 $P$ 映到射线 $O P$ 上一点 $P'$,且满足:
$
|O P||O P'| = r^2
$
]
#proposition[][
- 反演变换满足:$phi^2 = I$
- 反演变换将不经过 $O$ 的圆映成圆,将经过 $O$ 的圆映成直线,将不经过 $O$ 点的直线映成圆
- 反演变换将与 $C$ 正交的圆,经过 $O$ 的直线保持不变(注:正交指两圆心与交点的连线垂直)
]
#proof[
+ 是显然的\
+ 设另一圆的方程为:
$
(X^T, 1) mat(I, beta;beta^T, d) vec(X, 1) = 0
$
反演变换即为:$X -> c/(X^T X) X$,从而圆的像的方程为:
$
(c/(X^T X) X^T, 1) mat(I, beta;beta^T, d) vec(c/(X^T X) X, 1) = 0\
(c X^T, X^T X) mat(I, beta;beta^T, d) vec(c X, X^T X) = 0\
d (X^T X)^2 + 2 c beta^T (X^T X)X + c^2 X^T X = 0\
d X^T X + 2 c beta^T X + c^2 = 0\
$
从而 $d = 0$ 时它就是直线,否则是圆:
$
X^T X + (2 c)/d beta^T X + c^2/d = 0\
$
同时,它就是原来的圆当且仅当:
$
c = d
$
+ 保持过 $O$ 直线不变是显然的,保持正交的圆参照上面的计算即可。
//(X - O)^T (X-O) = r^2 <=> X^T X - 2 O^T X + O^T O - r^2 = 0$,设其上一对对称点为 $X_1, X_2$,则有:
// $
// X_2 - O = r^2/((X_1 - O)^T (X_1 - O)) X_1 - O
// $
// 在反演变换下,圆 $C$ 变为:
// $
// (O^T O - r^2)X^T X - 2 c O^T X + c^2 = 0
// $
]
#remark[][
反演可被看作球极投影,也即找一个球与一个平面,将球上某点在平面上的投影和该点关于球极在平面上的中心投影相关联,这就是平面上的反演变换
]
#definition[扩充平面][
在 $EE^2$ 上增加一个无穷远点 $infinity$,在反演中补充将原点映为 $infinity$,此时反演是 $overline(EE^2)$ 上的一一对应。
]
#theorem[保角][
反演变换保持角度(这里指原来的直线夹角等于映射后圆的夹角),但方向相反
]
#proof[
从几何的角度来说,我们可以构造圆的夹角(某个交点处两个圆各自切线的夹角),然后证明圆的夹角不变即可)。\
选取交于点 $P$ 的两个圆,满足两条切线 $l_1, l_2$ 的夹角之间包含原来的角。\
经过反演变换,$l_1, l_2$ 变为过 $O$ 点的圆,且一个交点为 $sigma(P)$。\
可以证明,$phi(l_1), phi(l_2)$ (作为圆)在 $O$ 处切线与 $l_1, l_2$ 平行但反向,进而圆 $phi(l_1), phi(l_2)$ 的夹角与原来两个圆的夹角相等
]
== 用复数表示反演变换
人们发现,表示反演最好的方式是看作复平面(加上无穷远点)上的变换。加上一个无穷远点得到的平面称为黎曼面,记作 $overline(CC)$。\
#remark[][
复平面上任意一个圆的方程可以写作:
$
(z, 1)mat(I, -overline(z_0);z_0, d) vec(overline(z), 1) = 0\
<=>z overline(z) - z z_0 - overline(z) overline(z_0) + d = 0\
<=> (z - z_0) (overline(z - z_0)) = -d + z_0 overline(z_0)
$
其中圆心为 $z_0$,半径的平方为 $-d + z_0 overline(z_0)$\
换言之,设 $z_1$ 的反演像为 $z_2$ 当且仅当:
$
(z_1 - z_0)(overline(z_2 - z_0)) = -d + z_0 overline(z_0)
$
整理回去,这就是:
$
(z_1, 1)mat(I, -overline(z_0);z_0, d) vec(overline(z_2), 1) = 0\
$
换言之,只需要在圆的方程中,将 $z$ 换为 $z_1$,将 $overline(z)$ 换为 $overline(z_2)$ 即可\
]<关于圆的反演>
#proposition[][
假设反演中心为 $z_0$,半径为 $r$,则有变换公式:
$
phi(z) = z_0 + r^2/(overline(z) - overline(z_0))
$
若关于以原点为中心的单位圆做反演,则得到更简单的公式:
$
phi(z) = 1/overline(z)
$
]
#definition[关于圆的对称点][
设 $z_1$ 关于圆 $C$ 的反演像为 $z_2$,则称 $z_1, z_2$ 关于圆 $C$ 对称
]
#proposition[][
反演保持两点关于圆的对称性
]
#proof[
不妨设反演是关于圆心在原点的单位圆上的反演\
设圆 $C$ 的方程为:
$
f(z, overline(z)) = 0
$
则它的反演像为:
$
f(Inv(phi)(z), Inv(phi)(overline(z))) = 0\
<=> f(1/overline(z), 1/z) = 0
$
我们只需要验证 $z_1, z_2$ 的像 $phi(z_1), phi(z_2)$ 在新的圆上保持对称即可,也即:
$
0 = f(1/overline(phi(z_1)), 1/phi(z_2)) = f(z_1, overline(z_2)) = 0
$
(这是因为@关于圆的反演 中的论断)\
从而结论成立
]
在黎曼面上,我们往往认为一条直线包含无穷远点是一个无穷大圆,因此将圆和直线做以统一的看待,同时将关于直线的反射称为关于无穷大圆的反演。
#theorem[][
关于直线的反射可以表示为 $sigma_1 dot sigma_2 dot sigma_1$,其中 $sigma_1, sigma_2$ 是关于普通圆的共轭。
]
== 莫比乌斯变换
#definition[莫比乌斯变换][
$overline(CC)$ 上偶数个关于圆的反演称为莫比乌斯变换,奇数个称为反莫比乌斯变换。记所有莫比乌斯变换/反莫比乌斯变换构成的集合为 $M_2^+, M_2^-$,并令 $M = M_2^+ union M_2^-$。$M_2, M_2^+$ 构成群,且 $|M_2 : M_2^+| = 2$,进而成为正规子群。
]
#proposition[][
平面上常见的变换,包括平移、旋转、位似可以唯一扩充为复平面上的莫比乌斯变换
]
#theorem[][
扩充复平面上的莫比乌斯变换可以表示为分式线性变换:
$
sigma(z) = (a z + b)/(c z + d)\
(a d - b c) != 0, a, b, c, d in CC
$
反莫比乌斯变换可以表示为共轭分式线性变换,也即:
$
sigma(z) = (a overline(z) + b)/(c overline(z) + d)\
(a d - b c) != 0, a, b, c, d in CC
$
且这些表示方式都是唯一的。
]
#proof[
+ 容易验证分式线性变换的复合还是分式线性变换,只需证明两个反演的复合是分式线性变换即可。
+ 只需证明分式线性变换与反演的复合是共轭分式线性变换即可
+ 对于唯一性,容易验证两个系数不同的分式线性变换一定表示不同的变换
]
#proposition[分式线性变换的代数表示][
设所有分式线性变换构成的群为 $H$,则有:
$
H tilde.eq quotient("SL"(2, CC), {I, -I}) := "PSL"(2, CC)
$
]
#theorem[][
扩充复平面上的分式线性变换可以表示为正伸缩变换和反定向保距变换的复合,或者是反演变换和保定向保距变换的复合。
]
#theorem[][
任何三点确定一个(反)莫比乌斯变换
]
#proof[
只需证明给定任何三点 $z_1, z_2, z_3$,存在唯一(反)莫比乌斯变换将三点变为:
$
0, 1, infinity
$
这是简单的。\
- 假如三点不共线,关于过三点的圆做反演使得三点共线
- 关于 $z_3$ 为圆心,取适当半径使得 $z_1, z_2$ 的像的距离为 $1$(由连续性这是可行的)
- 再加上平移和旋转便可让三点的像满足要求
注意最后我们反演的次数可能为奇数或偶数,这是无关紧要的,只需要再做一次共轭(关于实轴的反演)即可。
]
#theorem[][
(共轭)分式线性变换与(反)莫比乌斯变换之间存在一一对应,同时它们都被不同三点的像唯一确定
]
#proof[
对于任意分式线性变换,考虑 $0, 1, infinity$ 三点的像确定的(反)莫比乌斯变换,这个莫比乌斯变换的分式线性表示当然就是原来的分式线性变换。由于表示的唯一性,这也是唯一的满足要求的莫比乌斯变换,因此一一对应性成立。\
同时,三点的像唯一确定一个分式线性变换的容易验证的,进而也唯一确定一个莫比乌斯变换。
]
#proposition[][
分式线性变换 $f(z) = (a z + b)/(c z + d)$ 是正伸缩与保定向保距变换的复合,或者反演与反定向保距变换的复合\
共轭分式线性变换是反定向保距,或者正伸缩与直线反射,或者反演与保定向保距变换的复合
]
#proof[
只证明前者,后者是类似的
- 若 $c = 0$,则:
$
f(z) = (a/d) z + (b/d)
$
令 $a/d = r e^(i theta)$ ,这当然是正伸缩与保定向保距变换的复合
- 若 $c != 0$,不妨设 $a d - b c = 1$,取 $z_0 = phi(infinity) = a/c$,我们想先处理无穷点,从而取圆心为 $a/c$,半径为 $r$ 的反演 $phi_1$,计算得:
$
phi compose f(z) = - (overline(c))/c overline(z) + (overline(a) - overline(d))/c
$
这当然是一个反定向的保距变换
]
#corollary[][
任何莫比乌斯变换可以写成至多四个反演变换的复合。
]
#example[保持两点不动的莫比乌斯变换][
- 设莫比乌斯变换保持两点 $a, b$ 不动,则:
$
(sigma(z) - a)/(sigma(z) - b) = r e^(i theta)(z - a)/(z - b)
$
且 $r, theta$ 与 $z$ 无关。
- 进一步,设 $sigma(z_1) = infinity, sigma(infinity) = z_2$,将有:
$
r e^(i theta)(z_1 - a)/(z_1 - b) = 1\
(z_2 - a)/(z_2 - b) = r e^(i theta)\
$
从而 $z_1, z_2$ 同时成为二次方程:
$
(x-a)/(x-b) + (x-b)(x-a) = r e^(i theta) + 1/r e^(-i theta)
$
的两个根
]
#definition[复交比][
定义复平面上互异四点的交比为:
$
(A, B;C, D) = ((A - C)/(A - D)) / ((B - C)/(B - D))
$
它在莫比乌斯变换下保持不变,在反莫比乌斯变换下变为它的共轭
]
#theorem[][
复平面上四点共圆(包括无穷大圆)当且仅当四点交比为实数
]
#proof[
注意到反莫比乌斯变换与复交比的性质,若四点共圆,则关于此圆做反演可得四点交比等于自己的共轭。\
若四点不共圆,取适当的莫比乌斯变换将 $z_1, z_2, z_3$ 变为 $0, 1, infinity$,同时 $z_4$ 此时不是实数(否则它们共无穷大圆),计算验证四点交比不是实数即可
]
== 双曲几何
=== 圆盘模型
#definition[庞卡莱的双曲几何模型][
令 $D = {z in CC | |z| < 1}$。无穷远点定义为 ${z | |z| = 1}$\
变换群 $H$ 为所有保持 $D$ 稳定(集合不动)的莫比乌斯变换,这被称为双曲几何变化群。\
定义直线为所有与无穷远圆 $D$ 正交的圆或直线 $Tau$,关于 $Tau$ 的反演将保持 $D$ 不变,两个反演的复合被称为双曲变换。\
定义双曲反射是关于(上面定义的)直线的反演变化,它保持 $D$ 不变。\
(注意到莫比乌斯变换保持角度,因此保持正交,故将双曲直线映为双曲直线)
]
#proposition[][
$H$ 中任何一个变换可表示为两个双曲反射的复合
]
#proof[
取 $Z_0, Z_1$ 使得 $phi(Z_0) = 0, phi(Z_1) = 1$\
再设 $Z_0^*$ 为 $Z_0$ 关于 $D$ 的对称点。由于莫比乌斯变换保持圆的对称点不动,因此有:
$
phi(Z_0^*) = infinity
$
- 若 $Z_0 = 0$, 则 $phi$ 保持 $0, infinity$ 不动,这表明 $phi$ 就是平凡的旋转,也就是两个过 $O$ 点直线反演的复合
- 若 $Z_0 != 0$,则 $Z_0^* != infinity$\
此时,先作以 $Z_0^*$ 为圆心与 $D$ 正交的圆,将关于这个圆的反演记为 $phi_1$,则有:
$
phi_1 (D) = D\
phi_1 (Z_0^*) = infinity\
phi_1 (Z_0) = 0 "(这是因为保持对称点不动)"
$
此时当然有 $phi_1(Z_1) != infinity$,故取 $phi_1 (Z_1), phi(Z_1)$ 的中垂线。注意到 $phi_1 (Z_1)$ 仍在圆上,这个中垂线当然过点 $0$\
记关于这条中垂线的反射为 $phi_2$,我们发现:
$
phi_2 compose phi_1 (Z_0, Z_1, Z_0^*) = (0, 1, infinity) = phi(Z_0, Z_1, Z_0^*)
$
由于莫比乌斯变换的像由不同三点唯一确定,因此我们有:
$
phi_2 compose phi_1 = phi
$
证毕
]
#proposition[][
任取 $z_1 in D, z_2 in S_infinity$,存在双曲变换使得:
$
phi(z_0) = 0\
phi(z_1) = 1
$
]
这个命题说明双曲平面上任何一点都没有特殊之处。\
#proposition[几何公理][
- 过任何两点存在唯一双曲直线
- 过 $l$ 外一点 $C$,存在无穷多条与 $l$ 不交的双曲直线
]
#proof[
+ 不妨设 $a = 0$,结论是容易的(注意到反演将过原点 $0$ 的圆映成直线,将与 $D$ 正交的圆保持不动,因此过 $0$ 的圆不可能与 $D$ 垂直)
+ 同样不妨设 $c = 0$,此时 $l$ 是一个与 $D$ 正交的圆,当然容易取出无穷多条过 $0$ 的直线与 $l$ 不交
]
#proposition[][
任给圆周 $D$ 上三点组 $z_1, z_2, z_2$, $w_1, w_2, w_3$,存在至多三个双曲反射它们的复合 $phi$ 满足:
$
phi(z_1, z_2, z_3) = (w_1, w_2, w_3)
$
]
#proof[
显然这样的莫比乌斯变换是存在且唯一的,记为 $phi$,同时 $phi$ 一定会保持圆周不变
- 若 $z_1, z_2, z_3$ 与 $w_1, w_2, w_3$ 旋转方向相同,则由交比知保持圆周的内部,进而保持圆内集合稳定。因此 $phi in H$,进而是至多两个双曲反射的复合。
- 若 $z_1, z_2, z_3$ 与 $w_1, w_2, w_3$ 旋转方向相反,再取双曲反射将 $w_1, w_3$ 互换即可
]
#theorem[][
任何圆盘 $D$ 上的莫比乌斯变换可表示为:
$
phi(Z) = e^(i theta)(z-a)/(overline(a)z - 1), |a| < 1
$
反莫比乌斯变换可表示为:
$
phi(Z) = e^(i theta)(overline(z)-a)/(overline(a)overline(z) - 1), |a| < 1
$
]
#definition[庞加莱圆盘上的双曲距离][
在庞加莱圆盘上,对任意点 $z_1, z_2$,按照如下方法定义距离:\
过 $z_1, z_2$ 作唯一的双曲直线 $l$ 交圆周 $D$ 于 $eta_1, eta_2$,使得 $eta_1, z_1, z_2, eta_2$ 逆时针排列。则定义:
$
d(z_1, z_2) = -ln ((z_2, z_2;eta_1, eta_2))
$
(注意到由于四点共线,复交比是实数)
为 $z_1, z_2$ 间的双曲距离,同样也是:
$
ln (|z_1 - eta_2| |z_2 - eta_1|)/(|z_1 - eta_1| |z_2 - eta_2|)
$<distance>
]
#lemma[][
$p(0, z) = ln (1 + |z|)/(1 - |z|)$
]<distance-to-zero>
这个性质表明定义的距离与无穷远圆是相容的
#proposition[][
双曲距离满足:
- 正定性: $d(z_1, z_2) >=0, d(z_1, z_2) = 0 <=> z_1 = z_2$
- 对称性: $d(z_1, z_2) = d(z_2, z_1)$
- 在双曲反射,双曲变换下保持不变
- 若 $z_1, z_2, z_3$ 三点共线,且 $z_2$ 在 $z_1, z_3$ 之间,则:
$
p(z_1, z_2) + p(z_2, z_3) = p(z_1, z_3)
$
同时满足三角不等式,但证明略微复杂,我们留到后面
]
#proof[
+ 由定义,不难看出 $|z_1 - eta_2| >= |z_2 - eta_2|, |z_2 - eta_1| >= |z_1 - eta_1|$,因此在@distance 中自然有 $p(z_1, z_2) >=0$,同时等于零当且仅当 $z_1 = z_2$
+ 显然
+ 由于双曲反射,双曲变换保持圆周 $D$,保持复交比(保持复交比进而保持复交比的正负,从而顺序也得以保持),结论显然
+ 取双曲变换,不妨设 $z_2 = 0$,进行计算可得
]
#proposition[][
$p(z, w) = ln (|overline(z) w - 1| + |z - w|)/(|overline(z) w - 1| - |z-w|)$
]
#proof[
取双曲反射:
$
sigma(x) = z/(overline(z)) (overline(x) - overline(z))/(z overline(x) - 1)
$
它将 $z$ 变成零,利用 @distance-to-zero 计算即可
]
#proposition[][
- $cosh^2 (1/2 p(z, w)) = (|1 - z overline(w)|^2)/((1 - |z|^2)(1-|w|^2))$
- $sinh^2 (1/2 p(z, w)) = (|w - z |^2)/((1 - |z|^2)(1-|w|^2))$
- $tanh^2 (1/2 p(z, w)) = (|w - z |^2)/(|1 - z overline(w)|^2)$
]
#proposition[][
设点 $x$ 到原点 $o$ 的欧式距离为 $r$,则双曲距离满足:
$
p(0, x) = ln (1 + r)/(1-r) > r
$
同时,注意到当 $r -> 0$ 时上式与 $r$ 是同阶无穷小,意味着假如最开始的圆盘取的充分大,或者说考虑的尺度非常小,则双曲几何近似于欧式几何
]
#corollary[][
双曲圆也是欧式圆
]
#proposition[三角不等式][
双曲距离满足三角不等式:
$
p(z_1, z_2) + p(z_2, z_3) >= p(z_1, z_3)
$
取等当且仅当 $z_2$ 位于线段 $z_1 z_3$ 上
]
#proof[
#lemma[双曲直角三角形中,斜边大于直角边][
设 $A in l$, 过 $B$ 作垂直于 $l$ 的双曲直线 $l'$ 交 $l$ 于 $M$(注意到我们可以把 $A$ 映到原点,从而证明这样的直线是存在的)\
则有:
$
p(A, M) <= p(A, B)
$
取等当且仅当 $B in l$
]
#proof[
不妨设 $A$ 是原点,$l$ 是实轴,则取 $B$ 关于 $l$ 的对称点 $B'$,过 $B, B'$ 的双曲直线 $l'$ 一定与 $l$ 垂直\
注意到欧式圆也是双曲圆,从图形上容易看出结论成立
]
回到命题的证明,只需过 $z_2$ 作直线 $l$ 垂直 $z_1, z_3$,结合引理和之前证明的可加性容易得到结论成立。
]
#proposition[双曲正/余弦定理][
记 $A B C$ 为一个双曲三角形,对应边分别为 $a, b, c$,三个角分别为 $A, B, C$\
我们有:
- 双曲正弦定理:$(sinh a)/(sin A) = (sinh b)/(sin B) = (sinh c)/(sin C)$
- 双曲余弦定理:$cosh c = cosh a cosh b - sinh a sinh b cos C$
- 对偶余弦定理:$- cos C = cos A cos B - cosh c sin A sin B $
这表明双曲几何中,同样只有全等没有相似
]
#proof[
先证明双曲余弦定理,不妨设 $C = 0$,则:
$
a = ln (1+r)/(1-r)\
b = ln (1+s)/(1-s)\
c = ln (1+delta)/(1-delta), delta = (|s - r|)/(|r s - 1|)
$
同时:
$
r = tanh (a/2)\
s = tanh (b/2)\
delta = tanh (c/2)
$
注意此时的 $cos C$ 就是欧式几何中的 $cos C$ 因此可以利用欧式几何中的余弦定理,计算即得。\
对于正弦定理,我们只需利用余弦定理计算平方之后化简即可。\
由正弦定理,设:
$
sin A = R sinh A ...
$
带入计算即得对偶余弦定理
]
#remark[][
回忆 @球面几何正余弦 中球面几何的正余弦公式,它们几乎是一致的,只是将边的正余弦换成了双曲正余弦
]
#proposition[][
半径为 $r$ 的双曲圆的周长为 $2 pi sinh(r)$
]
=== 上半平面模型
在圆盘模型中,如果取 $i$ 为圆心,半径为 $sqrt(2)$ 的圆做反演变换。这个反演把圆盘 $D$ 映为下半平面。再取关于实轴的反演,便将下半平面映为上半平面。这样,我们就得到了上半平面模型。\
或者说,在莫比乌斯变换:
$
phi(z) = (-i z + 1)/(z - i)
$
下,单位圆盘被映成上半平面。\
#definition[上半平面模型][
将上述的莫比乌斯变换下 $D$ 的像成为上半平面模型,其上的距离自然的定义为拉回圆盘模型上的双曲距离。\
双曲直线共有两类,包括与实轴垂直的上半直线与圆心在实轴上的上半圆
同时,假设圆盘模型的双曲变换群为 $H$,当然有上半平面模型中,保持上半平面不变的所有莫比乌斯变换恰为:
$
phi compose H compose Inv(phi)
$
]
显然,上半平面模型与圆盘模型等距同构。但在某些应用中比庞卡莱模型更加方便一些。\
#proposition[][
设 $x = a + b i, y = a + c i, c > b$,则:
$
p(x, y) = ln (c/b)
$
]
#proof[
通过平移和放缩,不妨设 $x = im, y = lambda i, lambda = c/b$,进而:
$
Inv(phi)(x) = 0\
Inv(phi)(y) = (1-lambda)/(i+lambda i)
$
在庞卡莱圆盘模型中,计算距离即得结论。
]
#proposition[][
- @distance 中距离计算公式同样成立
-
$
p(z, w) = ln (|z - overline(w)| + |z - w|)/(|z - overline(w)| - |z - w|)
$
]
#definition[双曲面积][
(若积分存在)定义上半平面模型中某个区域 $A$ 的面积为:
$
integral_A 1/y^2 d x d y
$
]
#definition[双曲多边形][
由 $n$ 段双曲线段组成的闭曲线称为双曲多边形,两个双曲线段的交点称为顶点,在无穷远点的顶点称为理想点。\
]
#theorem[Gauss - Binet][
设双曲三角形 $A B C$ 的三个角分别为 $A, B, C$,则三角形的面积为:
$
pi - A - B - C
$
]
#proof[
- 假设至少有一个理想点,不妨设两边为垂直实轴的直线,计算积分即可
- 若不然,将 $A B C$ 延伸至有理想点的三角形 $A B D$,则有:
$
S(A C D) = pi - A - angle A C D\
S(C B D) = pi - angle C B D - angle D C B = B - angle D C B\
$
两式相减即可
]
#theorem[海伦公式][
设双曲三角形 $A B C$ 三边为 $a, b, c$,令:
$
alpha = cosh(a), beta = cosh(b), gamma = cosh(c)\
Delta = sqrt(1 - alpha^2 - beta^2 - gamma^2 + 2 alpha beta gamma )
$
则:
$
cos(S) = (alpha + beta + gamma + alpha beta + beta gamma + alpha gamma + alpha^2 + beta^2 + gamma^2 -alpha beta gamma )/((1+alpha)(1+beta)(1+gamma))\
tan(S/2) = Delta/(1 + alpha + beta + gamma)\
sin(S/2) = Delta/(4 cosh(a/2)cosh(b/2)cosh(c/2))
$
]
#proof[
证明可见:\
https://www.maths.gla.ac.uk/wws/cabripages/hyperbolic/hareaproof.html
]
#remark[][
双曲几何中未必三点一定确定一条直线或一个圆,例如 $-2 + i, i, 2 + i$ 不在任何双曲直线或圆上
]
#proposition[][
上半平面模型中,所有双曲变换可表示为:
$
phi(z) = (a z + b)/(c z + d), a d - b c = 1, a, b, c, d in RR\
phi(z) = (a overline(z) + b)/(c overline(z) + d), a d - b c = 1, a, b, c, d in RR
$
进一步,有:
$
Isom(UU) tilde.eq "PSL"(2, RR) times ZZ_2
$
]
#definition[][
取 $T != I in "PSL"(2, RR)$,$"Tr"(T) = |a + d|$:
- 若 $"Tr"(T) < 2$,$T$ 被称为椭圆的,它共轭于 $z -> e^(i theta) z$,恰有一个不动点
- 若 $"Tr"(T) = 2$,$T$ 被称为抛物的,它共轭于 $z -> z + 1$,没有不动点
- 若 $"Tr"(T) > 2$,$T$ 被称为双曲的,它共轭于 $z -> lambda^2 z, lambda > 1$,有两个不动点
]
#proof[
考虑 $"PSL"(2, RR)$ 中元素的相似分类即可
]
#definition[][
任取 $T in "PSL"(2, RR)$ 为双曲的,设其不动点为 $z_1, z_2$,则称 $T$ 的轴为过 $z_1, z_2$ 的双曲直线,显然这条直线被 $T$ 保持不变
]
#definition[][
为 $"PSL"(2, RR)$ 上附上 $RR^4$ 上的通常度量(距离定义为所有 $|T-S|$ 取正负中较小的)和拓扑,则乘积和取逆都是连续的,意味着构成一个拓扑群。
]
=== 射影洛伦兹模型
#definition[射影洛伦兹模型][
在线性空间 $RR times RR^2$ 上定义内积:
$
vec(x_1, y_1) dot vec(x_2, y_2) = x_1 x_2 - y_1^T y_2
$
等价于在 $RR^3$ 上定义内积:
$
alpha dot beta = alpha^T mat(1, 0, 0;0, -1, 0;0, 0, -1)beta
$
其中 $x$ 称为时间分量,$y$ 称为空间分量。这个内积不具有正定性。\
满足 $alpha dot alpha = 0$ 的向量称为光锥,$alpha dot alpha > 0$ 称为类时向量,$alpha dot alpha < 0$ 称为类空向量\
在这个内积下,定义:
$
F = {x in RR^3 | norm(x)^2 = 1, x_1 > 0}
$
对 $x, y in F$,定义:
$
d(x, y) = arccos (x dot y)
$
它的合法性由下面的引理给出
]
#lemma[][
设 $x, y$ 是正类时向量,则:
$
(x dot y)^2 >= norm(x)^2 norm(y)^2
$
]
#proof[
设 $V = generatedBy(x\, y)$ ,洛伦兹内积在其上是实对称的非正定/负定的双线性函数。注意到:
$
((x, y) X) dot ((x, y) Y) = X^T vec(x^T, y^T) mat(1, 0, 0;0, -1, 0;0, 0, -1) (x, y) Y \
= X^T mat(x dot x, x dot y; x dot y, y dot y) Y
$
由于非正定非负定,故系数矩阵行列式小于 $0$,这就是原结论
]
#theorem[][
$
funcDef(phi, F, D, (x_1, x_1, x_2), (x_1 + i x_2)/(1 + x_0))
$
是双曲平面的等距映射
]
这个事实并不平凡,证明在此略过
#definition[][
在洛伦兹空间中,定义叉乘为仿照欧式空间的叉乘(同样将后两项的符号取反)
]
#proposition[][
洛伦兹叉乘有类似一般叉乘的代数性质
]
#theorem[][
洛伦兹空间上的一个保距变换一定是线性变换,同时满足:
$
S mat(1, 0, 0;0, -1, 0;0, 0, -1) S^T = mat(1, 0, 0;0, -1, 0;0, 0, -1),\
|S| = 1
$
]
#lemma[][
设 $A B C$ 是双曲三角形,边长为 $a b c$ ,则:
$
cosh(c) = A dot B\
sinh(c) = -i norm(A times B)\
cos(C) = - (C times A) dot (C times B)/(norm(C times A) norm(C times B))
$
]
有了这些公式,我们将可以利用类似球面几何的向量计算的方法计算三角公式的正确性。
一般的,我们定义:
#definition[双曲几何][
一个度量空间称为 $2$ 维双曲几何,如果它与圆盘模型/上半平面模型等距同构
]
= 拓扑空间
== 概念
拓扑空间可以想成 $RR^n$ 上良好的开/闭结构的一种推广
#definition[拓扑][
$S$ 被称为一个拓扑空间,如果:
- 存在集合族 $Tau$,其中元素称为开集
- $emptyset in Tau$
- 任意多个开集的并仍是开集
- 有限多个开集的交仍是开集
此时,称 $Tau$ 为 $S$ 上的一个拓扑。也称开集的补集为闭集。
]
#proposition[][
闭集满足:
- 任意多个闭集的交仍是闭集
- 有限多个闭集的并仍是闭集
]
#example[][
任何度量空间都可以仿照欧式情形定义拓扑,称为欧式拓扑。特别的,离散度量给出的拓扑中,每个点都是开集,称为离散拓扑。
]
#definition[邻域][
称一个点的 $X$ 的任意包含一个包含 $X$ 的开集的开集为邻域
]
#definition[聚点/极限点][
称 $A$ 是集合 $E$ 的的极限点,如果对于 $A$ 的任意邻域 $X$,均有 $X sect E$ 非空
]
#remark[][
类似可以定义序列的极限点,但是在一般拓扑空间中序列可能有多个极限点,同时集合的极限点也未必是其中某个序列的极限点,因此不是很常用
]
#theorem[][
- $X$ 是闭集当且仅当它包含所有聚点。
- 聚点的聚点还是原集合的聚点
由此,称 $X$ 并上所有极限点称为 $X$ 的闭包,它是最小的包含 $A$ 的闭集
]
#definition[连续映射][
- 称 $f: A -> B$ 是连续映射,如果对任意开集 $S subset B$ 均有 $Inv(f)(S)$ 是开集
- 称 $f: A -> B$ 在 $x_0$ 处连续,如果 $f$ 在 $x_0$ 的某个邻域连续
]
#proposition[][
- 函数在 $X$ 上连续当且仅当在 $X$ 中每个点连续
- 连续映射的复合还是连续的
]
#proof[
+ 一方面是显然的,另一方面,假设 $f$ 在每个点上连续,从而对每个点 $x$,存在 $S_x$ 使得 $f|_(S_x) : S_x -> B$ 连续。任取开集 $Y subset B$,将有:
$
A = union.big_(x in A) S_x\
Inv(f)(Y) = union.big_(x in A) S_x sect Inv(f)(Y) = union.big_(x in A) Inv(f|_(S_x))(Y)
$
由题设及开集的并仍是开集知原命题成立
]
#theorem[][
下列说法等价
- 映射连续
- 映射满足闭集的原像是闭集
- 对于任意 $Beta$ 是 $Y$ 的拓扑基,也即任何 $Y$ 中开集都可写作 $Beta$ 中某些元素的并,映射满足拓扑基中开集的元素是开集
- $forall X subset A$,有 $f(overline(A)) subset overline(f(A))$
- $forall Y subset B$,有 $Inv(f)(overline(A)) subset overline(Inv(f)(A))$
]
#lemma[][
设 $A_i$ 是有限个闭集,且 $X = union.big A_i$。若 $f$ 在每个 $A_i$ 上都连续,则 $f$ 在 $X$ 上连续
]
#remark[][
这个引理改为有限/无穷多个开集仍然成立,但是无穷个闭集是不行的
]
#example[乘积拓扑][
设 $S, T$ 为拓扑空间,则 $S times T$ 上的拓扑定义为由 $S$ 中开集与 $T$ 中开集相乘作为拓扑基生成的拓扑。可递归定义有限多情形。对于无穷情形,我们一般要求其中至多有限个不是全集。
]
事实上,我们规定的乘积拓扑就是满足两个投影函数均连续的最小拓扑,因此自然满足下面的定理:
#theorem[][
$f: Z -> X times Y$ 连续当且仅当 $p_x compose f, p_y compose f$ 都连续
]
#example[][
设 $X = RR union {0^+}$,其中开集为 $RR$ 中开集或者 $(-a, 0) union (0, b) union {0^+}$。在这个拓扑空间中,$0, 0^+$ 的任何邻域都是相交的,因此这不可能是一个度量空间。这种拓扑空间是不可分的,略显病态。
]
== 分离与可数
#definition[分离性][
设 $X$ 为拓扑空间:
- 称 $X$ 为 $T_1$ 空间,若对任何 $x != y$,存在 $x, y$ 的邻域使得其中不包含另外一个(一个等价的说法是单点集是闭集)
- 称 $X$ 为 $T_2$ 或者 Hausdoff,如果对任何 $x != y$,存在 $x, y$ 的邻域使得两者不相交
- 称 $X$ 为 $T_3$ (正则,Regular)的,如果它是 $T_1$ 的且点与不相交的闭集有不相交的开邻域。一个等价说法是 $T_1$ 且对 $forall x$ 及它的开邻域 $W_1$,都存在开邻域 $U$ 使得:
$
X in U subset overline(U) subset W
$
- 称 $X$ 为 $T_4$ (正规, Normal)的,如果它是 $T_1$ 的且不相交的闭集之间有不相交的开邻域, 一个等价说法是 $T_1$ 且对 $forall "闭集" A$ 及它的开邻域 $W_1$,都存在开邻域 $U$ 使得:
$
A subset U subset overline(U) subset W
$
以上四者依次增强
]
#proposition[][
- 在 $T_1$ 空间中,若 $X$ 是 $A$ 的聚点,则 $X$ 的任意邻域都与 $A$ 交于无穷多个点
- 在 Hausdoff 空间中,一个序列至多有一个极限点
- $X times Y$ 是 Hausdoff 空间当且仅当 $X, Y$ 都是 Hausdoff 的
- 度量空间是 $T_1, T_2, T_3, T_4$ 的
]
#definition[可数性][
- 称 $X$ 为第一可数或者 $C_1$,若 $forall x in X$ 有可数多个邻域基,也即存在可数多个邻域
- 若 $A subset X$ 满足 $overline(A) = X$,则称 $A$ 在 $X$ 中稠密。若 $X$ 有可数多稠密的基,则称 $X$ 是可分的
- 称 $X$ 为第二可数或者 $C_2$ 若 $X$ 有可数多的拓扑基
以上三者依次增强
]
#theorem[Lindeluf][
$C_2 + T_3 => T_4$
]
#proof[
取 $X$ 的可数多拓扑基 $BB = {B_1, B_2, ..., B_n, }$,对与任意不相交闭集 $A_1, A_2$\
令 $BB_i$ 为闭包与 $A_i$ 不交的所有 $B$ 的集合,再令:
$
U_n = B_2^n - union.big overline(B_2^i)\
V_n = B_1^n - union.big overline(B_1^i)
$
取 $U = union U_i, V = union V_i$,则 $U sect V = emptyset$\
任取 $x in A_1$,由 $T_3$ 知 $X$ 与 $A_2$ 有不相交的开邻域 $W_x, W_x'$,满足存在开集 $B$, $x in B subset W_x$\
而 $overline(B) sect A_2 = emptyset => B = "某个" B_n$\
$A_1 sect union B_2^i = emptyset => x in U_n subset U => A_1 subset U, A_2 subset V$
]
#proposition[][
可分的度量空间是第二可数的
]
#lemma[][
对 $T_4$ 空间中,若 $A sect B = emptyset$,则存在连续函数 $f$ 使得:
$
f(A) = 0, f(B) = 1
$
反之,若这个条件恒成立,则空间一定是 $T_4$ 的
]
#proof[
这个构造是很难的,思路类似于戴德金分划\
令 $QQ_0 = QQ sect [0, 1] = {r_1 = 1, r_2 = 0, r_3, r_4, ...}$\
对 $r_i$ 构造开集 $U_i$,满足:
- $r_n < r_m => overline(U_n) subset U_m$
- $A subset U_n subset B^c$
定义函数 $f(x) = sup{0, r_n in QQ_0 | x in.not U_n} = inf{1, r_n in QQ_0 | x in U_n}$,只要证明它是连续函数即可
]
#theorem[Tiltzet][
设 $X$ 是 $T_4$ 空间,$A$ 是其中的闭集,$f: A -> R$ 是连续函数,则 $f$ 可被连续的延拓到 $X$ 上\
同时,若此结果成立,容易验证空间一定是 $T_4$ 的
]
#proof[
我们的思路是找一串一致收敛的函数\
不妨设 $f(A) subset [-1, 1]$(否则取 $arctan f := g$ 再做处理即可)。\
令 $A_1 = Inv(f)([-1, -1/3]), B_1 = Inv(f)([1/3, 1])$\
显然 $A_1, B_1$ 是 $X$ 中不相交的闭集,由之前的引理,将存在连续函数 $phi$ 使得:
$
phi_1(A_1) = - 1/3, phi_1(B_1) = 1/3
$
令 $f_1 = f - phi$,不难发现 $f_1(A) subset [-2/3, 2/3]$\
反复进行这个步骤,不难得到一串函数 $phi_i subset [- (2^(n-1))/3^n, (2^(n-1))/3^n]$,利用维尔斯特拉斯判别法将其求和即可
]
#definition[可度量化][
称一个拓扑空间可度量化,如果存在一个度量,它诱导的拓扑空间与原空间同构
]
#lemma[Vrysohn][
$C_2 + T_4 =>$可度量
]
== 紧致与列紧
#definition[紧致][
- 称一个拓扑空间是紧致的,如果其上任何开覆盖都有有限开覆盖。
- 称一个拓扑空间是列紧的,如果其上任何无穷序列都有收敛子列。
]
#theorem[][
紧致空间的无穷子集必有聚点\
注:这并不意味着紧致一定列紧,它们之间是互不包含的关系
]
#lemma[Lebesgue][
设 $X$ 是紧致度量空间,$e$ 是开覆盖,则存在 $delta > 0$,使得 $X$ 的任意直径小于 $delta$ 的子集都包含在 $e$ 的某一个开集之内,$delta$ 被称为 $e$ 的 Lebesgue 数
]<Lebesgue-lemma>
#theorem[][
- 紧致集的连续像仍然紧致
- 紧致集的闭子集仍然紧致
- 列紧空间上的实值函数有界且可取得最大最小值
- 度量空间中紧致等价于列紧
- $X times Y$ 紧致当且仅当 $X, Y$ 都紧致
]
#proof[
- 不妨假设 $f: X -> Y$ 是满连续映射,$X$ 是紧致集。\
设 $BB$ 是 $Y$ 的一个开覆盖,则:
$
"map"_Inv(f) BB = {Inv(f)(B) | B in BB}
$
当然是一个 $X$ 的开覆盖,它将有有限开覆盖:
$
AA' subset "map"_Inv(f) BB
$
此时 $Y = f(X) subset union.big "map"_f AA' := BB' subset BB$
- 设 $U$ 是 紧致空间 $X$ 的闭子集,设 $BB$ 是其一个开覆盖,则 $BB union {X - U}$ 当然也是 $X$ 的开覆盖,它将有有限开覆盖 $BB'$。\
$BB'$ 当然也覆盖 $U$,且要么是 $BB$ 中有限个覆盖,要么是这样有限个覆盖加上 $X - U$。前者结论已成立,后者去掉 $X - U$ 不会影响它是 $X$ 的覆盖,进而结论也成立
- 同 $RR$ 上情景的证明
- 一方面,在度量空间中可以构造一个邻域开球,这个开球不包含之前任意有限多的点; 另一方面,由 @Lebesgue-lemma, 我们想到构造一些点,这些点的 $delta$ 邻域足以覆盖整个 $X$,进而在引理中取这些点对应的开集即可。事实上这些点是可以取得的,否则我们将可以得到一个无穷序列,其中每两个元素的距离都大于 $delta$,但这和列紧的定义无疑是矛盾的
- 一方面,由 $X times Y -> X, Y$ 的投影映射是连续的,当然有紧致集的连续像紧致。另一方面,我们有引理: \
假设 $A subset X$ 紧致,$y in Y$, $W$ 是 $X times Y$ 中紧致集 $A times {y}$ 的开邻域,则存在 $A$ 的开邻域 $U$ 与 $y$ 的开邻域 $V$ 使得 $W subset U times V$
]
#remark[][
在欧式拓扑中,紧致当且仅当是有界闭集,但在一般拓扑空间中未必。例如假设 $X$ 多于两个元素,赋予平凡拓扑仅有 $X, emptyset$ 为空集,此时单点集当然紧致但不闭。同时,一个集合紧致,它的闭包也未必紧致。
]
#theorem[Tychonoff][
设 $X_alpha, alpha in omega$ 是任意多的非空的紧致空间,则所有这些集合构成的乘积拓扑是紧致的
]
#proof[
它的证明是较为复杂的,我们不证明。事实上,它与选择公理是等价的。
]
#theorem[][
Hausdoff 空间的紧子集都是闭集
]
#proof[
设 $A$ 是紧子集,$y in X - A, forall x in A$ ,存在不交的开邻域 $y in U_x, x in V_x$\
取遍所有 $x$, $V_x$ 将构成一个开覆盖,它有有限开覆盖,记这些开覆盖的对应的 $U$ 的交为 $BB_y$,它是与 $A$ 不交的开集\
不难发现 $X - A = union.big_(y in - A) BB_y$ 是开集,因此结论成立
]
#theorem[][
紧致空间到 Hausdoff 的可逆连续映射一定是同胚
]
#proposition[][
$X$ Hausdoff, $A, B$ 紧致且不相交,则它们有不相交的开邻域
]
== 连通性
#definition[连通][
称 $X$ 是连通的,如果它不能写成不交的非空开子集之并
]
#theorem[][
设 $X$ 可被若干连通子集 $A_i$ 覆盖,且 $sect.big A_i$ 非空,则 $X$ 连通
]<连通判别法>
#proof[
反证法,假设 $X = U_1 union U_2$,其中 $U_1, U_2$ 是开集。\
由于 $sect.big A_i$ 非空,不妨设 $sect.big A_i subset U_1$,则 $U_2$ 与 $sect.big A_i$ 不交,
]
#theorem[][
$X$ 是连通的当且仅当其中既闭又开的集合只有空间和全集
]
#definition[道路/道路连通][
- 称 $[0, 1] -> X$ 的连续映射为一条道路,$f(0)$ 为起点,$f(1)$ 为终点。显然首尾相接的道路可以连接起来,称其为 $f, g$ 的衔接 $f . g$(先走 $g$ 再走 $f$)
- 称 $X$ 道路连通,若对任何两点 $x, y in X$,存在 $x -> y$ 的道路
]
#proposition[][
道路连通的空间连通
]
#proof[
由 @连通判别法,任取 $x_0 in X$,对所有 $y$ 构造 $U_y$ 为 $x -> y$ 的道路 $f$ 对应的 $f([0, 1])$\
显然 $U_y$ 满足定理条件,因此结论成立。
]
#theorem[][
欧式空间的连通开集是道路连通的
]
#proof[
设 $U$ 是欧式空间的连通开集,$U'$ 是其中道路连通的子集。显然它不是空集,我们希望证明它既是开集也是闭集,因此是全集。
- 首先证明 $U'$ 是开集。事实上,取 $x$ 是其中一个点,取一个小开球 $B$ 使得 $B subset U$。断言 $U' union B$ 也是道路连通的,这是因为 $B$ 之中,$U'$ 之中的两点都是互相道路连通的,而对于各自两点,总可找到各自到 $x$ 道路,将它们连接起来即可。
- 再证明它是闭集。设 $y in U$ 是 $U'$ 的聚点,同样取 $y$ 的小开球 $B$ 使得 $B subset Y$,显然其中存在 $U'$ 中的点 $z_0$,将 $y -> z_0$ 道路与 $z_0 -> x$ 道路复合即得 $y -> x$ 道路,因此 $y in U'$,结论成立。
这就表明 $U'$ 既开又闭,因此是全集。
]
#example[][
设 $X = {(0, y) | -1 <= y<= 1}, U = {(x, sin 1/x) | 0 < x <= 1}$,则 $X union U$ 是连通集但不道路连通
]
== 粘合空间
#definition[][
设 $X$ 是拓扑空间,$P$ 是其不交划分。构造拓扑空间:
$
Y = P
$
显然存在满映射 $pi: X -> Y$\
取使得 $f$ 连续的最大拓扑,也即 $O subset Y$ 是开集当且仅当 $Inv(pi)(O) = union.big O$ 是开集。\
或者将 $Y$ 视作某个等价关系下的等价类,结果是相同的。\
这个拓扑称为粘合拓扑或者商拓扑,空间称为商空间
]
#theorem[][
设 $Y$ 是商空间,$pi : X -> Y$ 是自然映射,$f: Y -> Z$ 是到拓扑空间 $Z$ 的映射,则:
$
f "连续" <=> f compose pi "连续"
$
]
事实上,这也唯一刻画的商拓扑的性质,作为推论,商映射的复合仍是商映射
#theorem[][
划分的加细可以诱导出商空间上的商空间,而这个空间在同构意义下与划分的顺序无关。事实上,这就是第三同构定理
]
#definition[][
设 $f$ 连续
- 若 $f$ 保持开集,则称其为开映射
- 若 $f$ 保持闭集,则称其为闭映射
容易验证开/闭映射的复合仍然是开/闭映射,它们都是商映射,但反之未必
]
#theorem[][
紧致空间到 Hausdoff 的连续满射必为商映射
]
期末考试:1月12日 8:30 - 10:30,理教 206
答疑:1月 2, 4, 9, 11 智华楼473
|
|
https://github.com/Myriad-Dreamin/typst.ts | https://raw.githubusercontent.com/Myriad-Dreamin/typst.ts/main/fuzzers/corpora/math/style_02.typ | typst | Apache License 2.0 |
#import "/contrib/templates/std-tests/preset.typ": *
#show: test-page
// Test forcing math size
$a/b, display(a/b), display(a)/display(b), inline(a/b), script(a/b), sscript(a/b) \
mono(script(a/b)), script(mono(a/b))\
script(a^b, cramped: #true), script(a^b, cramped: #false)$
|
https://github.com/shunichironomura/ukaren-typst-template | https://raw.githubusercontent.com/shunichironomura/ukaren-typst-template/main/README.md | markdown | # 宇宙科学技術連合講演会予稿集原稿用Typstテンプレート
宇宙科学技術連合講演会の予稿集原稿をTypstで作成するためのテンプレートを含むレポジトリです。
## [`template.typ`](template.typ)
[予稿集原稿投稿ページ](https://smartconf.jp/content/ukaren68/abstructionupload)およびそこからダウンロード可能な予稿集原稿テンプレートをもとに筆者が作成したテンプレートです。
## [`example.typ`](example.typ)
テンプレートを用いて、公式に提供されている[予稿集原稿テンプレート(Wordファイル)](https://pcojapan-online.com/uploads/Paper_Sample68.docx)を再現するための例です。出力は[`example.pdf`](example.pdf)を参照してください。
## 公式テンプレートとの差異
現状、作成要領に反しない範囲ですが、少なくとも以下の点で公式テンプレートとの差異があります。
- 日本語フォント
- 公式:MS明朝
- このテンプレート:游明朝(MS明朝ではセクションタイトルを太字にできないため)
- 参考文献の書式
- 公式:`著者名:文献タイトル, 誌名, 巻, 号, 頁, 年.`
- このテンプレート:`著者名. 文献タイトル. 誌名. 年, 巻, 号, 頁` ([SIST02](https://warp.ndl.go.jp/info:ndljp/pid/12003258/jipsti.jst.go.jp/sist/handbook/sist02_2007/main.htm)書式)
|
|
https://github.com/qujihan/typst-cv-template | https://raw.githubusercontent.com/qujihan/typst-cv-template/main/utils.typ | typst | #import "params.typ": *
#let icon-text-box(
icon-path,
txt,
size: 16pt,
baseline: 3.5em,
h-size: 0.5em,
icon-factor: 1.2,
) = {
box(baseline: baseline, outset: (x: 0pt))[#image(
project-root + icon-path,
height: size * icon-factor,
)]
h(h-size)
box(outset: (y: 0pt), height: auto)[#text(size: size)[#txt]]
}
#let emphasize(color: default-config.emphasize-color, content) = {
highlight(
fill: color,
top-edge: 0.2pt,
bottom-edge: -1.5pt,
content,
)
}
#let boldline(v-size: default-config.bold-line-space) = {
block(
below: 0pt,
above: 0pt,
{
v(v-size * 0.1)
line(length: 100%, stroke: (paint: black, thickness: 0.8pt))
v(v-size * 0.9)
},
)
}
#let thinline(v-size: default-config.thin-line-space) = {
v(v-size * 0.3)
line(
length: 100%,
stroke: (paint: black, dash: ("dot", 5pt, 5pt, 5pt)),
)
v(v-size * 0.7)
}
#let display-cv-info(
info,
name-size: default-config.head-info-name-size,
other-size: default-config.head-info-other-size,
name-color: default-config.head-info-name-color,
other-color: default-config.head-info-other-color,
) = {
let block-size = name-size + other-size * 2 + 1em
block(above: 0em, below: 2em, height: block-size, width: 100%)[
#set align(center + horizon)
#set text(fill: name-color, weight: "bold", name-size)
#info.name
#set align(center + bottom)
#set text(fill: other-color, weight: "regular", other-size)
#box(
align(center)[
#info.email #info.wechat
],
)
#linebreak()
#box(
align(center)[
#info.phone
],
)
]
}
#let show-string-arr-one-line(str-arr) = {
let len = str-arr.len()
columns(len, gutter: 0%)[
#for i in range(len) {
if i == 0 {
align(left)[#str-arr.at(i)]
colbreak()
} else if i == len - 1 {
align(right)[#str-arr.at(i)]
} else {
align(center)[#str-arr.at(i)]
colbreak()
}
}
]
}
#let section-title(icon-path, content) = {
block(
above: 2em,
below: 0.5em,
{
set text(weight: 500, fill: black)
icon-text-box(
icon-path,
content,
size: 1.3em,
baseline: 0.2em,
h-size: 0.5em,
icon-factor: 1,
)
v(0.4em)
boldline()
},
)
}
#let subsection-list(str-arr) = {
set text(1.1em, weight: 400, fill: black)
show-string-arr-one-line(str-arr)
}
|
|
https://github.com/QuadnucYard/pigeonful-typ | https://raw.githubusercontent.com/QuadnucYard/pigeonful-typ/main/README.md | markdown | MIT License | # Pigeonful
Are you dreaming of becoming a pigeon? Now let's start!
This is a typst package for creating splendid document similar to [研招网推免](https://yz.chsi.com.cn/tm/student/dlqtz/list.action) style. Have fun!
## Examples
All of the examples below derive from web images.
### KFC

```typst
#import "../src/lib.typ": pigeonful
#set page(width: auto, height: auto, margin: 4pt)
#pigeonful(
entries: (
"层次": [个人练习生],
"单位": [加里敦大学],
"院系": [肯德基学院],
"专业": [疯狂星期四工程(Vme50)],
"学习方式": [全日制],
"研究方向": [吮指原味鸡烹饪自动化],
"厨师": [不区分厨师],
"专项计划类型": [非专项计划],
"就业类型": [非定向就业],
),
notifier: [加里敦大学 招生办 2022-09-28 12:42],
notice-body: [加里敦大学肯德基学院已在教育部推免服务系统中完成待录取通知发送工作,请您尽快在推免服务系统中确认录取。逾期未确认者,将视为放弃我院的待录取资格。],
acceptance: [您于9月28日 12:44接受了加里敦大学的待录取通知],
width: 810pt,
)
```
### National Holiday

```typst
#import "../src/lib.typ": pigeonful
#set page(width: auto, height: auto, margin: 4pt)
#pigeonful(
entries: (
"我也": [不知道],
"发生": [了什么],
"但是": [听说],
"在今天": [只要发这种配色的图片],
"大家就算": [不会点开看],
"也会有": [很多人点赞],
"我不信": [所以来试试],
),
notifier: [国庆假期 招生办 2024-09-29 10:23],
notice-body: [尊敬的同学:您好,感谢您选择国庆假期。距离国庆还有一天,今天是调休日,要一起快乐起来吗?],
acceptance: [你于9月29日 10:31接受了国庆假期的待录取通知],
width: 768pt,
)
```
## Usage
See examples. You just need to fill in the relevant arguments of the `pigeonful` function.
You may need a `Microsoft YaHei` font installment.
|
https://github.com/binhtran432k/ungrammar-docs | https://raw.githubusercontent.com/binhtran432k/ungrammar-docs/main/contents/literature-review/esbuild.typ | typst | #import "/components/glossary.typ": gls
== ESBuild
<sec-esbuild>
ESBuild is a relatively new JavaScript bundler that has gained significant
attention due to its exceptional performance and innovative approach. We will
explore the key features, benefits, and challenges of using ESBuild for
bundling JavaScript applications.
ESBuild has emerged as a powerful and efficient bundler for modern JavaScript
applications. Its exceptional performance, focus on simplicity, and growing
community support make it a compelling choice for developers seeking to
optimize their build processes. While there may be some limitations to
consider, ESBuild's strengths significantly outweigh the challenges. As the
ESBuild ecosystem continues to evolve, it is likely to become an even more
popular and indispensable tool for JavaScript development @bib-esbuild.
=== Key Features
- *Bundling*: ESBuild efficiently combines multiple JavaScript modules into a
single file for optimized delivery.
- *Minification*: Automatically minifies the bundled code, reducing its size
and improving load times.
- *Tree Shaking*: Eliminates unused code, further optimizing bundle size and
performance.
- *Source Maps*: Generates source maps for debugging purposes, enabling easier
navigation between the original code and the bundled output.
- *Plugin Support*: Extensible through plugins, allowing for customization and
integration with other tools.
- *TypeScript Support*: Built-in support for TypeScript, including type
checking and transpilation.
=== Performance and Speed
- *Parallel Processing*: ESBuild leverages parallel processing to significantly
speed up the bundling process, especially for large projects.
- *Incremental Builds*: Optimizes rebuild times by only recompiling affected
modules when changes are made.
- *Efficient Algorithms*: Employs efficient algorithms for code analysis and
optimization, resulting in faster bundling and smaller output sizes.
|
|
https://github.com/WinstonMDP/math | https://raw.githubusercontent.com/WinstonMDP/math/main/exers/e.typ | typst | #import "../cfg.typ": *
#show: cfg
$ "Prove that" (RR, +), (RR without 0, *) "aren't isomorphic groups" $
Suppose the opposite
$(RR without 0, *) tilde.eq (RR_+, *)$
$1 = phi(1) = phi(-1^2) = phi(-1) phi(-1)$
$phi(-1) = 1 = phi(1)$
$bot$
|
|
https://github.com/jgm/typst-hs | https://raw.githubusercontent.com/jgm/typst-hs/main/test/typ/compiler/string-08.typ | typst | Other | // Error: 2-21 string index -1 is not a character boundary
#"🏳️🌈".slice(0, -1)
|
https://github.com/jneug/schule-typst | https://raw.githubusercontent.com/jneug/schule-typst/main/src/ab.typ | typst | MIT License | #import "_imports.typ": *
#let arbeitsblatt(
..args,
body,
) = {
let (
doc,
page-init,
tpl,
) = base-template(
type: "AB",
type-long: "Arbeitsblatt",
//_tpl: (:),
..args,
body,
)
{
show: page-init
tpl
}
}
|
https://github.com/OrangeX4/typst-sympy-calculator | https://raw.githubusercontent.com/OrangeX4/typst-sympy-calculator/main/tests/test.typ | typst | MIT License | #import "cal.typ": *
// set variances
#let a = 1
#let b = $a + 1$
|
https://github.com/Lypsilonx/boxr | https://raw.githubusercontent.com/Lypsilonx/boxr/main/README.md | markdown | MIT License | # Boxr
Boxr is a modular, and easy to use, package for creating cardboard cutouts in Typst.
## Usage
Create a boxr structure in your project with the following code:
```
#import "@preview/boxr:0.1.0": *
#render-structure(
"box",
width: 100pt,
height: 100pt,
depth: 100pt,
tab-size: 20pt,
[
Hello from boxr!
]
)
```
The `render-structure` function is the main function for boxr. It either takes a path to one of the default structures provided by boxr (e.g.: `"box"`) or an unpacked json file with your own custom structure (e.g.: `json("my-structure.json")`). These describe the structure of the cutout.\
The other named arguments depend on the structure you are rendering. All unnamed arguments are passed to the structure as content and will be rendered on each box face (not triangles or tabs).
## Creating your own structures
Structures are defined in `.json` files. An example structure that just shows a box with a tab on one face is shown below:
```
{
"variables": ["height", "width", "tab-size"],
"width": "width",
"height": "height + tab-size",
"offset-x": "",
"offset-y": "tab-size",
"root": {
"type": "box",
"id": 0,
"width": "width",
"height": "height",
"children": {
"top": "tab(tab-size, tab-size)"
}
}
}
```
The `variables` key is a list of variable names that can be passed to the structure. These will be required to be passed to the `render-structure` function.\
The `width` and `height` keys are evaluated to calculate the width and height of the structure.\
The `offset-x` and `offset-y` keys are evaluated to place the structure in the middle of its bounds. It is relative to the root node. In this case for example, the top tab adds a `tab-size` on top of the box as opposed to the bottom, where there is no tab. So this `tab-size` is added to the `offset-y`.\
`root` denotes the first node in the structure.\
A node can be of the following types:
- `box`:
- The root node has a `width` and a `height`. All following nodes have a `size`. Child nodes use `size` and the parent node's `width` and `height` to calculate their own width and height.
- Can have `children` nodes.
- Can have an `id` key that is used to place content on the face of the box. The id-th unnamed argument is placed on the face. Multiple faces can have the same id.
- Can have a `no-fold` key. If this exists, no fold stroke will be drawn between this box and its parent.
- `triangle-<left|right>`:
- Has a `width` and `height`.
- `left` and `right` denote the direction the other right angled line is facing relative to the base.
- Can have `children` nodes.
- Can have a `no-fold` key. If this exists, no fold stroke will be drawn between this triangle and its parent.
- `tab`:
- Is not a json object, but a string that denotes a tab. The tab is placed on the parent node.
- Has a tab-size and a cutin-size inside the `()` separted by a `,`.
- `none`:
- Is not a json object, but a string that denotes no node. This is useful for deleting a cut-stroke between two nodes.
Every string value in the json file (`width: "__", height: "__", ... offset-x/y: "__"` and the values between the `()` for tabs) is evaluated as regular typst code. This means that you can use all named variables passed to the structure. All inputs are converted to floats (/ pt) and the result of the evaluation will be converted back to a length.
|
https://github.com/icpmoles/politypst | https://raw.githubusercontent.com/icpmoles/politypst/main/aside/appendix_B.typ | typst | #let app_B = [
#heading(numbering: none)[Appendix B]
It may be necessary to include another appendix to better organize the presentation of
supplementary material.
] |
|
https://github.com/giZoes/justsit-thesis-typst-template | https://raw.githubusercontent.com/giZoes/justsit-thesis-typst-template/main/resources/layouts/appendix.typ | typst | MIT License | #import "@preview/i-figured:0.2.4"
#import "../utils/custom-numbering.typ": custom-numbering
// 后记,重置 heading 计数器
#let appendix(
numbering: custom-numbering.with(first-level: "", depth: 4, "1.1 "),
// figure 计数
show-figure: i-figured.show-figure.with(numbering: "1-1"),
// equation 计数
show-equation: i-figured.show-equation.with(numbering: "(1-1)"),
// 重置计数
reset-counter: false,
it,
) = {
set heading(numbering: numbering)
if reset-counter {
counter(heading).update(0)
}
// 设置 figure 的编号
show figure: show-figure
// 设置 equation 的编号
show math.equation.where(block: true): show-equation
it
} |
https://github.com/kyliancc/mathematics | https://raw.githubusercontent.com/kyliancc/mathematics/main/Infomation%20and%20Entropy/cpt1/cpt1.typ | typst | #set page("a4")
#align(left, text(25pt)[
*Information and Entropy*
])
#align(left, text(20pt)[
*Chapter 1: Bits*
])
*The bit, is the fundamental unit of information.*
We use a common understanding *code*, which are sequence of 0 and 1, to convey information. For an instance, we use 0 and 1 to describe each side of a coin. In this case, when $n$ coins are flipped, the amount of outcomes is $2^n$, and we need $n$ *bits* to describe it.
There's some important things about information:
+ Information can be learned through observation, experiment, or measurement.
+ Information is subjective, or “observer-dependent”
+ A person's uncertainty can be increased upon learning that there is an observation about which information may be available, and then can be reduced by receiving that information
+ Information can be lost, either through loss of the data itself, or through loss of the code
+ The physical form of information is localized in space and time. As a consequence,
- Information can be sent from one place to another
- Information can be stored and then retrieved later
= The Boolean Function
Let me show some often used boolean functions with two input variables and one output value: AND, OR, XOR, NAND, and NOR:
$
N O T & space overline(A) \
A N D & space A dot B \
N A N D & space overline(A dot B) \
O R & space A + B \
N O R & space overline(A + B) \
X O R & space A plus.circle B
$
|
|
https://github.com/WinstonMDP/math | https://raw.githubusercontent.com/WinstonMDP/math/main/knowledge/residue_fields.typ | typst | #import "../cfg.typ": cfg
#show: cfg
= Residue classes
$a equiv_n b := n divides a - b$.
$[a]_n := {x in ZZ mid(|) x equiv_n a}$.
A residue ring $a$ modulo $n := ZZ_n := ZZ op(slash) equiv_n$.
$ZZ_n$ is an associative commutative ring with a unit.
$ZZ_n$ is a field $<-> n$ is prime.
$[z]_n$ is invertible in $ZZ_n <-> "GCD"(n, z) = 1$.
*Fermat's little theorem*: $x^p equiv_p x$.
$(p - 1)! equiv_p -1$.
|
|
https://github.com/MichaelFraiman/TAU_template | https://raw.githubusercontent.com/MichaelFraiman/TAU_template/main/README.md | markdown | # Readme
## What is this?
This is a template used to produce ODE materials for semester א of the year ה׳תשפ׳׳ד at TAU.
Apart from the style files, one actual use-case file is shared.
## What state is it in?
Raw.
The design has been adapted to the fonts which are not shared here (Meta Serif, Helvetica).
Although, you can probably use Google Noto Fonts (create a `fonts` folder and put them there);
you need also Hebrew and Arabic Noto.
Currently there is not much customisation available.
It was also tuned to just the English language and headers in Hebrew and Arabic.
Bidirectional typesetting is currently messed up, but I am working on it.
## How to use?
Compile and watch with `compile.py` and `watch.py`.
You can do `python3 compile.py file`, where `file` can be `.typ` or `.pdf` (or just with a trailing period or without it).
## Note
1. This file will probably be updated retroactively, as it is a copy of the working-directory file.
2. Currently Typst is unstable, so beware of the future changes (I think you need v.0.11 to compile this).
3. Features will be added in the future.
|
|
https://github.com/Sepax/Typst | https://raw.githubusercontent.com/Sepax/Typst/main/DIT323/Assignments/A3/main.typ | typst |
#import "template.typ": *
#show: template.with(
title: [Finite automata and formal languages #linebreak() Assignment 3],
short_title: "DIT084",
description: [
DIT323 (Finite automata and formal languages)\ at Gothenburg University
],
authors: ((name: "<NAME>"),),
lof: false,
lot: false,
lol: false,
paper_size: "a4",
cols: 1,
text_font: "XCharter",
code_font: "Cascadia Mono",
accent: "#000000", // black
)
=
Let $Sigma = {0,1}$
Let $R & = (0 + 01^*)^* (epsilon + 1) 1 (epsilon + 0 + 1)^*$
Let's construct a NFA for R:
+ Construct a NFA for $(0 + 01^*)^*$
#figure(image("figures/F6.jpg", width: 50%))
+ Add $(epsilon + 1) 1$ to the NFA
#figure(image("figures/F7.jpg", width: 50%))
+ Add $(epsilon + 0 + 1)^*$ to the NFA
#figure(image("figures/F8.jpg", width: 50%))
The NFA for R is the thus following:
#sourcecode[```
0 1
→ q0 {q0 q1} {q2 q3}
q1 {} {q0 q1}
q2 {} {q3}
* q3 {q3} {q3}
```
]
#pagebreak()
=
The regular expression is: $R = 1(1+01)^+$
- The "$1$" in the beginning makes it so that the string must start with a $1$.
- The "$+$" makes it so $|w| gt.eq 2$
- $(1+01)$ makes it so it always ends with a $1$ and two zeroes never appear after
one another.
=
Let the following table represent the NFA:
#table(
columns: (auto, auto, auto, auto),
inset: 10pt,
align: horizon,
[*State*], [$epsilon$], [$a$], [$b$],
[$-> s_0$], [$emptyset$], [$s_1$], [${s_0,s_2}$],
[$ s_1$], [$s_2$], [$s_4$], [$s_3$],
[$s_2$], [$emptyset$], [${s_1, s_4}$], [$s_3$],
[$s_3$], [$s_5$], [${s_4,s_5}$], [$emptyset$],
[$s_4$], [$s_3$], [$emptyset$], [$s_5$],
[$"*" s_5$], [$emptyset$], [$s_5$], [$s_5$]
)
We then define the following DFA by subset construction:
#table(
columns: (auto, auto, auto),
inset: 10pt,
align: horizon,
[*State*], [$a$], [$b$],
[$-> s_0$], [${s_1,s_2}$], [${s_0, s_2}$],
[${s_1,s_2}$], [${s_1,s_2,s_3,s_4,s_5}$],[${s_3,s_5}$],
[${s_0, s_2}$], [${s_1,s_2,s_3,s_4,s_5}$], [${s_0,s_2,s_3,s_5}$],
[$"*"{s_1,s_2,s_3,s_4,s_5}$], [${s_1,s_2,s_3,s_4,s_5}$], [${s_3,s_5}$],
[$"*"{s_3,s_5}$],[${s_3,s_4,s_5}$], [{$s_5$}],
[${s_0,s_2,s_3,s_5}$], [${s_1,s_2,s_3,s_4,s_5}$],[${s_0,s_2,s_3,s_5}$],
[$"*"{s_3,s_4,s_5}$],[${s_3,s_4,s_5}$],[$s_5$],
[$s_5$], [$s_5$], [$s_5$]
)
#pagebreak()
=
Transition diagram for the NFA:
#figure(image("figures/F9.jpg", width: 70%))
Let's define the following equations for the NFA:
$ q_0 &= epsilon + q_0 a \
q_1 &= q_0 b + q_2 b \
q_2 &= q_0 c + q_1 c \
q_3 &= q_1 b + q_3 c \
q_4 &= q_3 a + q_4 b + q_2 c $
The goal is to find the regular expression for the final state, $q_4$.
For $q_0$ we have:
$ q_0 &= epsilon + q_0 a & & \
&= epsilon a^* wide & & ("Arden's lemma") $
For $q_1$ we have:
$ q_1 &= q_0 b + q_2 b \
&= q_0 b + (q_0 c + q_1 c) b wide & & ("Substitute" q_2) \
&= q_0 b + q_0 c b + q_1 c b \
&= (q_0 b + q_0 c b) (c b)^* wide & & ("Arden's lemma") $
For $q_2$ we have:
$ q_2 &= q_0 c + q_1 c \
&= q_0 c + (q_0 b + q_0 c b) (c b)^* c wide & & ("Substitute" q_1) $
#pagebreak()
For $q_3$ we have:
$ q_3 &= q_1 b + q_3 c \
&= q_1 b c^* $
Now we solve $q_4$:
$ q_4 &= q_3 a + q_4 b + q_2 c & \
&= (q_3 a + q_2 c) b^* & ("Arden's lemma") \
&= ((q_1 b c^*) a + (q_0 c + (q_0 b + q_0 c b) (c b)^* c) c) b^* & ("Substitute" q_3 "and" q_2 ) \
&= (q_1 b c^* a + q_0 c c + q_0 b (c b)^* c c + q_0 c b (c b)^* c c ) b^* & ("Simplify") \
&= (((q_0 b + q_0 c b) (c b)^*) b c^* a + q_0 c c + q_0 b (c b)^* c c + q_0 c b (c b)^* c c ) b^* & ("Substitute" q_1) \
&= (q_0 b (c b)^* b c^* a + q_0 c b (c b)^* b c^* a + q_0 c c + q_0 b (c b)^* c c + q_0 c b (c b)^* c c ) b^* & ("Simplify") \
&= (epsilon a^* b (c b)^* b c^* a + epsilon a^* c b (c b)^* b c^* a + epsilon a^* c c + epsilon a^* b (c b)^* c c + epsilon a^* c b (c b)^* c c ) b^* & ("Substitute" q_0) \
&= (a^* b (c b)^* b c^* a + a^* c b (c b)^* b c^* a + a^* c c + a^* b (c b)^* c c + a^* c b (c b)^* c c ) b^* & ("Remove" epsilon) \
&= a^* (b (c b)^* b c^* a + c b (c b)^* b c^* a + c c + b (c b)^* c c + c b (c b)^* c c ) b^* & ("Simplify") \
$
|
|
https://github.com/alikindsys/aula | https://raw.githubusercontent.com/alikindsys/aula/master/Core/packages.typ | typst | // Acronym manager
#import "@preview/acrostiche:0.3.1" as acrostiche
// Callouts
#import "@preview/gentle-clues:0.6.0" as gentle-clues
// Qr Codes
#import "@preview/cades:0.3.0" as cades
// Fancy Headers and Footers
#import "@preview/chic-hdr:0.4.0" as chic-hdr
// Glossary
#import "@preview/gloss-awe:0.0.5" as gloss-awe
// Rust formatting & string interpolation
#import "@preview/oxifmt:0.2.0" as oxifmt
// Markdowm tables
#import "@preview/tablem:0.1.0" as tablem
// Custom table formatting
// See: https://github.com/PgBiel/typst-tablex?tab=readme-ov-file#reference
#import "@preview/tablex:0.0.8" as tablex
// Chemical typesetting
#import "@preview/whalogen:0.1.0" as whalogen
// Number, Units typesetting
#import "@preview/unify:0.4.3" as unify
// CeTZ
#import "@preview/cetz:0.2.0" as cetz
|
|
https://github.com/romero-kenny/Programming-Studio | https://raw.githubusercontent.com/romero-kenny/Programming-Studio/main/programming_studio.typ | typst | Creative Commons Zero v1.0 Universal | #import "./ieee-typst-template/template.typ": *
#show: ieee.with(
title: "Programming Studio: How Games Can Better Prepare Software Engineers",
abstract: [
// The complexity of modern software, built upon interconnected systems, presents a
// challenge for new software engineers. Traditional teaching methods often fail to
// develop the essential computational and systems thinking skills needed to
// navigate this complexity. We propose a solution inspired by game design: sandbox
// environments that encourage exploration, iterative practice, and intuitive
// learning. Unlike current curricula, which often focus on isolated
// problem-solving, these environments would empower students to apply their
// knowledge broadly, deepening their understanding of computer science and its
// real-world applications.
// The complexity of modern software presents a challenge to upcoming software
// engineers as computer science curricula does not equip students to effectively
// tackle large systems. This is due to computer science curriculum utilizing
// traditional teaching methods, rather than activity or project based methods
// to better challenge student's computational thinking. Games are a medium that
// foster computational thinking, as players understand the complexities of games
// as they play. Instructor can then take inspiration from game design in order to
// develop playful environments, which would foster deeper learning of content
// and develop computational thinking. This paper outlines a course that
// utilizes game design to foster a play environment specifically for computational
// thinking, and prepares students for future coursework and more complex software.
The complexity of modern software poses a significant challenge for aspiring
software engineers, as traditional computer science curricula often fall short
in preparing students to effectively navigate large systems. This gap arises
from the reliance on conventional teaching methods rather than engaging activity
or project-based approaches that could better stimulate students' computational
thinking. Games, serve as a medium that naturally cultivates computational
thinking, as players grapple with the intricacies of game mechanics while playing.
Drawing inspiration from game design principles, instructors can create playful
learning environments that promote deeper understanding of course content and
foster the development of computational thinking skills. This paper proposes
a course design that leverages game design to establish such a playful environment
specifically tailored for enhancing computational thinking, thereby equipping
students with the necessary skills for future coursework and tackling more
complex software projects.
],
authors: (
(
name: "<NAME>",
department: [Department of Computer Science],
organization: [University of North Georgia],
location: [Dahlonega, Georgia 30597],
email: "<EMAIL>"
),
),
index-terms: (
"active learning",
"project-based learning",
"activity-based learning",
"computational thinking",
"game design",
"play environment",),
bibliography-file: "../lpm.bib",
)
= Introduction
// #set quote(block: true)
// #quote(attribution: [<NAME>])[
// When you learn to read, you can then read to learn. And it’s the same thing
// with coding. If you learn to code, you can code to learn.
// ]
#set quote(block: true)
#quote(attribution: [<NAME>])[
I think that it’s extraordinarily important that we in computer science keep fun
in computing\[...\] Don’t feel as if the key to successful computing is only in
your hands. What’s in your hands, I think and hope, is intelligence: the ability
to see the machine as more than when you were first led up to it,
that you can make it more.
]
Current computer science curricula often fail to equip students with the skills
needed to address the complexity of today's software. An emphasis on memorization
hinders the development of computational thinking that is
essential for success. This approach forces students to repeatedly relearn material,
as they lack opportunities to apply their knowledge. Consequently, they are
ill-prepared for advanced courses that require programming experience and the
practical use of foundational concepts#cite(<follow_c>).
The primary obstacle is embedding computational thinking within the curriculum.
"Computational thinking involves solving problems, designing systems, and
understanding human behavior, by drawing on the concepts fundamental to computer
science."#cite(<comp_thinking>)
Programming, in turn, is the application of computational thinking. While curricula
centered on programming have been devised, they often prioritize correctness and tend to feature
relatively small-scale projects, thus failing to fully embrace computational thinking. To truly
cultivate computational thinking, there must be a shift towards larger-scale projects,
necessitating an educational environment conducive to project and activity based learning
#cite(<guide_teach_cs>).
Environments that promote exploration and confidence-building are needed#cite(<leisure_play>).
Classical teaching methods create environments that obscure the potential of
programming and limit exploration. This stifles students' sense of agency and discourages them
from exploring.
Minimizing the tools needed for programming empowers students by showing them they possess the
necessary resources to solve problems#cite(<guide_teach_cs>). Empowering students
to actively evaluate their understanding, iterate on their skills, and gain
confidence in their abilities#cite(<code_to_learn>).
This paper outlines methods for selecting tools and designing courses, illustrating
the creation of a curriculum that fosters computational thinking through a playful
environment.
= Creating A Play Environment
// #set quote(block: true)
// #quote(attribution: [<NAME>])[
// Some things are too important to be taken seriously
// ]
Video games are often misconstrued when it comes to their potential in education.
The concept of gamification has exacerbated this misunderstanding by framing games
solely as tools for enhancing engagement in the classroom. However, in reality,
games serve as exemplary environments for nurturing computational thinking skills
#cite(<jblow_vg_edu>).
Game developers intricately design complex systems that players must comprehend
to progress. Through the implementation of levels and mechanics, they construct
a series of incremental challenges that players must navigate. This approach
allows players to intuitively grasp the game's mechanics and continually test
and refine their understanding of its systems. Moreover, certain games, such
as _Kerbal Space Program_ for rocket science#cite(<kerbal>), _Minecraft_ for digital logic
#cite(<minecraft>), or
_Seven Billion Humans_ for algorithms (@seven_bil_humans), offer
immersive experiences that familiarize players with fundamental concepts rather
than directly teaching them#cite(<zachtronics>).
This immersion facilitates the development of a profound understanding of these
concepts at their core.
#figure(
image("./figures/seven_bil_humans.png"),
caption: [
A solution to a problem in Seven Billion Human using the least amount of code
]
) <seven_bil_humans>
Drawing inspiration from the structure of games, educators can design learning
environments that promote deeper comprehension of essential concepts. By
integrating game-like elements, such as incremental challenges and immersive
experiences, alongside traditional teaching methods, educators can foster
intuitive and active learning while addressing any misconceptions students
may have#cite(<guide_teach_cs>). Through careful selection of tools and the incorporation of playful
projects and problems, play environments can emulate the engaging nature of
games, enticing students to enjoy learning for its intrinsic value rather than
extrinsic rewards
#cite(<Moraz_n_2020>)
#cite(<game_development_teaching>)
#cite(<active_discrete_structs>).
== For the Student
Through the creation of playful learning environments, computational thinking
can flourish. Establishing a playful environment requires careful selection of
tools and assignments that empower students, fostering their agency and confidence.
This approach encourages exploration, experimentation, and iteration of knowledge
and skills. The choice of appropriate tools is dictated by the curriculum's
objectives. Just as game developers must adhere to the constraints of their genre
while innovating with mechanics and levels, instructors must similarly curate and
deploy tools and assignments that reinforce the core concepts of the curriculum.
This approach not only cultivates a deeper understanding of the subject-matter but
also nurtures an appreciation for it by giving rise to an intrinsic want to learn
#cite(<code_to_learn>)#cite(<jblow_vg_edu>).
Tools serve as vehicles for students to actively engage with the fundamental
concepts of the curriculum. Simplifying the complexity of these tools enables
students to direct their focus towards understanding the curriculum itself, rather
than grappling with the intricacies of the tools#cite(<jblow_vg_edu>) #cite(<zachtronics>).
When tools are easier to grasp, students are more inclined to believe in their
ability to master them, fostering a sense of agency and confidence.
// This empowerment
// encourages students to independently practice with the tools, thereby allowing
// more opportunities for students to gain a personal connection with the content
// and expand their understanding of the concept or computer science as a whole.
This empowerment fosters student autonomy in practicing with the tools, thereby
providing greater opportunities for students to develop a personal connection
with the content and deepen their understanding of the concept or computer science
as a whole.
As students develop a personal
connection with the material, they become motivated to explore the concepts more deeply,
leading to a heightened appreciation for them#cite(<code_to_learn>).
This intimate familiarity with the concepts
allows for the integration and interweaving of ideas, fostering a holistic
understanding of how the discipline operates as a cohesive system.
// Over time, this
// enables students to create a connected system of concepts for computer science;
// enabling them to continually expand their perspective of what can be done with computer
// science.
Over time, this empowers students to construct a cohesive framework of concepts
in computer science, enabling them to consistently broaden their perspective on the
possibilities within the field#cite(<guide_teach_cs>).
// Recognizing the diversity among students' ability, selecting appropriate assignments and
// challenges is paramount to nurturing computational thinking#cite(<guide_teach_cs>).
// Assignments should incorporate elements of exploration and iteration, compelling students to adopt a systemic perspective on how concepts interrelate. By iteratively building upon
// prior knowledge, students gradually gain confidence in their ability to practically
// apply these concepts, thereby empowering them with a sense of agency. The exploratory
// nature of such assignments encourages students to adopt an experimental approach,
// fostering an intuitive understanding of the underlying systems and deepening their
// comprehension. Activity and project-based assignments exemplify this exploratory
// and iterative approach, offering students opportunities to engage with tools in
// practical scenarios, even within simulated environments. Open-ended assignments
// further enable students to demonstrate their understanding and creativity, enhancing
// their confidence and sense of agency in the process. Through the iterative and
// exploratory nature of assignments, students experience growth in confidence and
// agency as they expand their knowledge and skills, allowing them to assess their
// progress by directly applying concepts. This dynamic also fosters a social dimension,
// as students are eager to share their discoveries with their peers.
Recognizing the diversity among students' abilities is key to nurturing computational
thinking#cite(<guide_teach_cs>). Assignments should encourage exploration
and iteration, fostering a systemic perspective on concepts. By building upon prior
knowledge and skills iteratively, students gain confidence and agency in applying these
concepts practically. This approach encourages experimentation, deepening comprehension
and fostering intuitive understanding#cite(<jblow_vg_edu>). Activity and project-based
assignments exemplify this approach, providing practical engagement within simulated
environments. Open-ended tasks allow students to demonstrate creativity and understanding,
instilling an intrinsic want to learn.
This iterative process promotes growth in confidence and skills,
facilitating direct application and fostering a social dimension as students share discoveries,
ideas, or designs with peers.
The social dimension of play cultivates community, empowering students and fostering
a culture within the environment. Just as games have clans and sports have clubs,
programming has its hacker communities#cite(<game_jams>)#cite(<kris_hackathons>).
Belonging to such communities provides
extrinsic motivation, as students find value in being part of a larger group.
Community membership also instills a sense of responsibility and sets expectations,
motivating students to explore and improve their skills#cite(<blow_depth_jam>).
// Play environments give rise to emergent properties, encouraging students to delve
// deeper into the underlying systems and continually refine their understanding.
// This not only promotes computational thinking but also allows students to develop
// other valuable soft skills essential in the workplace. By becoming more versatile
// individuals, students enhance their prospects for future employment or personal growth.
// Game developers allow the player to be rewarded for mastering and creatively
// using the environments and tools to solve challenges. Play environments can achieve
// this for instructors to allow for students to have a better understanding of the
// content and a better grasp with their tools. Instilling confidence and agency,
// while simultaneously developing computational thinking; empowering students
// for future coursework or opportunities.
// Game developers often reward players for mastering and creatively utilizing
// environments and tools to overcome challenges. Similarly, play environments in
// educational settings can achieve this by empowering students to better understand
// content and master their tools. By instilling confidence and agency, while simultaneously
// developing computational thinking skills, these environments prepare students
// for future coursework and opportunities.
Games developers craft an environment that instill an intrinsic want from the player to
continually better their skills and understanding of the game. Instructors can do
the same with play environments by utilizing activity and project-based assignments
and careful choice of tools. This intrinsic want cultivates a social aspect as
students want to share their ideas and skills to others.
== For the Instructor
// Instructors gain benefits from play environments. Just like a game developer
// receives feedback by watching players play or being a player themselves, instructors
// can do the same. Instructors are encouraged to partake in their own assignments
// and actively demonstrate their own skills in the curriculum being taught. Fostering
// an interswappable dynamic between student and instructor. Allowing the students
// to display their mastery, but also the instructor to learn from students to then
// better develop the course.
//
// Play environments benefit instructors. Game developer iterate on their game
// to improve it, to which they receive player feedback or are the player themselves.
// Play environments allow instructors to dynamically adjust the course to better
// suit the students skills, participate in the activity with them to either
// display a ceiling to reach or helping students struggling with the activity.
// Play allows for instructors to be creative with how they approach they're students,
// better engaging them in course, deepening their understanding, and able to learn
// from students, the same way a game developer does with their game.
Play environments offer significant benefits to instructors akin to how game
developers iterate on their games for improvement. Instructors can dynamically
adjust courses based on student skills, participating alongside them to set benchmarks
or assist those facing challenges. This flexibility allows instructors to creatively
engage students, enhancing their learning experience and deepening understanding.
Moreover, instructors can learn from students, much like game developers gather
feedback from players, leading to continuous improvement in course delivery and
effectiveness#cite(<guide_teach_cs>)#cite(<john_programming>).
// An activity-based approach give instructors free time, this free time should be
// used to further gauge the course, explore new technologies/tools, and/or help students.
// Gauging the course with free time is completing the assignment alongside the
// students, this can further be turned into a competition, or for the instructor
// to show off and obtain credibility with the students. The instructor can also
// try a new technology/tool when gauging the class to see if it complements the
// concepts for the curriculum better. This exploration can also inspire the instructor
// to gain new perspective on how to teach certain topics with the current tools in
// their disposal. Instructors can directly help students who are struggling
// with the activity/project, by either advice, diagrams, or demonstrations.
An activity and project based approach provides instructors with valuable free time #cite(<leisure_play>)#cite(<sep_plato>), which can be utilized to enhance the course in various ways. This time can be spent completing assignments alongside students, potentially turning it into a competition or an opportunity for instructors to demonstrate their expertise and build
credibility#cite(<guide_teach_cs>). Additionally, instructors can use this time to experiment with new technologies or tools to determine their suitability for the curriculum. This exploration can inspire instructors to gain fresh perspectives on teaching certain topics and
better engage students. Moreover, instructors can directly assist students who
are struggling with activities or projects by providing guidance, diagrams, or
demonstrations.
// Demonstrations are important unto themselves, as they give a tangible ceiling of
// how good a student can be. Though there may be better instructors or role models
// elsewhere, having a physically close demonstration is important in instilling
// culture, credibility and a personal connection with the students. By doing so,
// the instructor can more effectively teach students, as students are more open
// to the instructor's ideas. They allow students to understand the normalize
// way to approach their tools, or introduce them to new tools that will further
// help them in future coursework/career. Demonstrations can spark inspiration in
// students, allowing them to develop their understanding of the system that
// drives the discipline; or by creating a culture around certain topics/concepts,
// creating spaces that allow students to expand, challenge, and share their knowledge.
// If seeing is believing, demonstrations are key for students to see that they
// can achieve.
// Demonstrations play a crucial role as they provide a tangible benchmark for
// students to aspire to. Despite the existence of better instructors or role models
// elsewhere, close physical demonstrations are essential for instilling culture,
// credibility, and fostering personal connections with students. This closeness
// enables instructors to effectively convey ideas, with students being more receptive
// to their teachings. Demonstrations also help students understand the standard approach
// to using tools and may introduce them to new ones beneficial for future coursework
// or career advancement. Moreover, demonstrations can ignite inspiration in students,
// deepening their understanding of the subject matter and cultivating a culture
// around specific topics or concepts. Ultimately, demonstrations serve as powerful
// motivators for students, showing them that they too can achieve success.
// Activities allow for students to become the instructor, demonstrating their
// knowledge to the students and even to the instructor. This dynamic allows for the
// instructor to gauge the students' knowledge and to gain perspective on their
// view of the course and/or concepts being taught. This is necessary to better
// understand the effectiveness of the teaching methods implemented to teach the
// concepts for the course. This dynamic also allows the instructor to learn
// new perspectives from the students, giving the instructor more methods to teach
// students concepts of the curriculum, or to better connect with students.
// By allowing the student to become the instructor, it allows the instructor to
// better gauge their course and the effectiveness of their teaching methods; giving
// them a manner to dynamically alter the class to better suit the student's need
// to understand the material better.
Engaging in activities where students take on the role of the instructor enables
a dynamic exchange of knowledge#cite(<guide_teach_cs>)#cite(<john_programming>).
This allows instructors to assess students' understanding of the course material and gain
insights into their perspectives on the concepts being taught. Understanding the effectiveness of
teaching methods is essential for refining course delivery. Moreover, this approach empowers
instructors to learn new teaching methods from students, enhancing the instructor's ability
to convey curriculum concepts effectively and fostering stronger connections with
students. By facilitating this student-as-instructor dynamic, instructors can
better tailor the course to meet students' learning needs, ensuring a deeper
understanding of the material.
// Game developers utilize players to gauge the fun of their games, they may also
// be a player themselves to understand their game and instill skills, competition
// and community into the player base to allow them to gauge the game for them.
// Instructors can do the same through the activity and project based approaches
// that play environments incorporate, allowing them the ability to better cater
// the curriculum to the skills and understanding of the students, fostering
// a deeper understanding for more advanced coursework and simultaneously a community
// to allow further students to further develop their understanding with their peers and
// instructors.
Just as game developers engage players to assess game enjoyment and may also
play themselves to enhance skills and build community, instructors can adopt
similar approaches utilizing activity and project-based assignments. This
enables instructors to tailor the curriculum to students' skills and understanding,
fostering deeper comprehension of advanced coursework while nurturing a supportive
learning community where students can collaborate and develop their understanding
alongside peers and instructors.
= Course Design
// #show quote: set align(center)
// #quote(block: true, attribution: [Confucius])[
// I hear and I forget.
// I see and I remember.\
// I do and I understand.
// ]
// TODO: redo first paragraph to better expand idea, but also denote that this is not
// necessarily a new idea. We will just be extending the amount of time of such
// activities, allowing for a new manner to teach/design courses around
// computer science curricula. Cross fit for students, utilizing different
// methods to develop evenly develop their skills needed in computer science
// curricula or industry.
//
// The integration of game design into computer science curriculum has been previously
// explored. This paper draws inspiration from game jams, hackathons, and competitive
// programming, which leverage game design to cultivate creativity and competitiveness
// to motivate students, but also professionals to explore and learn new technologies/ideas.
// By creating a play environment with activities and projects inspired by these
// competitions, the aim is to develop computational thinking skills among students.
// This approach enables students to apply and refine their knowledge and skills,
// providing them with the necessary environment to enhance their abilities as
// software engineers and gain a systemic understanding of fundamental computer
// science curricula.
//
// The incorporation of game design into computer science education has been explored
// previously. This paper draws inspiration from events like game jams, hackathons,
// and competitive programming, which harness game design to foster creativity and
// competitiveness. These activities not only motivate students but also professionals
// to explore and learn new technologies and ideas. By establishing a playful
// environment with projects inspired by these competitions, the aim is to provide opportunities
// to develop computational thinking skills in students. This approach enables students
// to apply and refine their knowledge and skills, facilitating their growth as software
// engineers and providing a comprehensive understanding of fundamental computer
// science concepts.
//
// This course takes inspirations from <NAME>'s _Algorithmic Thinking_
// #cite(<algo_think>), <NAME>'s _CS 190: Software Design Studio_#cite(<john_cs190>),
// <NAME>'s course#cite(<follow_c>), hackathons#cite(<kris_hackathons>)
// and game jams#cite(<game_jams>) as the assignments
// utilize an activity and project based approach. As well as taking inspiration from
// <NAME>'s beginning years as a professional programmer#cite(<carmack>) to
// create the play environment, by having the students role-play as him.
//
// This course is sectioned into three sections. The first section is primary inspired
// by Zingaro's work#cite(<algo_think>), but instead utilizing competitive programming
// problems to practice and measure the student's proficiency with the programming.
// The second section is primarily inspired by hackathons,
// building confidence in students and also giving them a chance to practice with
// the library. The last section is primarily inspired by Ousterhout's course#cite(<john_cs190>)
// and game jams, utilizing the framework of Ousterhout's course and game jams'
// (more closely to <NAME>'s and <NAME>'s _Depth Jam_#cite(<jblow_depth_jam>))
// thematic project assignments to allow for an iterative work, feedback from peers
// and the instructor, while simultaneously allowing students to explore their own
// ideas and be creative. Lastly, utilizing a role-playing theme gives a fun purpose
// to the class, as students will mimic Carmack's life as he worked from contract programmer
// for monthly software to creating iD software.
//
This course draws inspiration from several sources: <NAME>'s _Algorithmic
Thinking_#cite(<algo_think>), <NAME>'s _CS 190: Software Design
Studio_#cite(<john_cs190>), <NAME>'s course#cite(<follow_c>),
hackathons#cite(<kris_hackathons>),
and game jams#cite(<game_jams>). The assignments follow an activity and project-based approach.
The course is divided into three sections. The first section, inspired by Zingaro's work
#cite(<algo_think>), focuses on competitive programming problems to assess students' programming
proficiency or teach a new language. The second section, inspired by
hackathons#cite(<kris_hackathons>), aims to build
students' confidence and provide practice with libraries. The final section draws inspiration
from Ousterhout's course#cite(<john_cs190>) and game jams#cite(<game_jams>), particularly
<NAME>'s and <NAME>'s _Depth Jam_#cite(<blow_depth_jam>), incorporating thematic
project assignments for iterative work and peer feedback. The third section encourages
exploration of ideas and creativity.
== Choosing a Programming Language and Library: Why Odin and Raylib?
// In truth, any language can be chosen as long as it fits the curriculum of the course
// or if utilizing the preferred language of the institution. However, one may want
// to adapt this course to also teach introductory programming or to transition
// to a different language#cite(<follow_c>). This course focuses on utilizing play to
// foster computational thinking, thus a simple, complete language is needed. _Python_,
// _Go_, and _C_ are great, as they have a rich ecosystem, ubiquitous and a simple syntax;
// allowing for an "easy to learn, hard to master" feeling, allowing students to do more
// with less and focus on implementation design to a problem.
//
// _Odin_#cite(<odin>) is a _C_ alternative with inspiration from _Go_ and
// _Python_#cite(<kris_odin>), it has a simple build system, easy interoperability with _C_, and a
// rich standard and vendor library. The base installation of _Odin_ is a battery included
// experience for new students, with a simple syntax for easy pickup. Allowing for a
// joyful experience programming with the power of _C_.
In truth, any language or library can be chosen as long as it aligns with the
course curriculum or the
institution's preferred language. However, one may wish to adapt this course to teach
introductory programming or transition to a different language #cite(<follow_c>).
This course focuses on
utilizing play to foster computational thinking, thus requiring a simple, comprehensive language.
_Python_, _Go_, and _C_ are excellent choices due to their rich ecosystems, ubiquity, and
simple, familar syntax, providing an "easy to learn, hard to master" experience that allows
students to accomplish more with less and concentrate on implementation design.
_Odin_#cite(<odin>) is a _C_ alternative inspired by _Pascal_, _Go_ and _Python_
#cite(<kris_odin>),
featuring a simple build system, easy
interoperability with _C_, and a rich standard and vendor library. The base installation of
_Odin_
provides a battery-included experience for new students, with a simple syntax for quick
learning. This enables a joyful programming experience with the power of _C_.
_Raylib_#cite(<raylib>) is simple library for putting graphics on the screen.
It was originally created for art students to understand the basics of game programming
#cite(<tj_raylib>). Written in _C99_, portable to different platforms and languages,
_Raylib_ is a great library to know for student's or instructor's own future tools,
projects or hobbies. The simplicity enables students to focus on creating their
ideas, whereas the portability allow students to explicitly take their skills
to other languages or platforms.
== Learning Odin through Advent of Code: 2 Weeks
// This section utilizes competitive programming problems to teach a new programming
// language. These problems are from _Advent of Code_#cite(<advent>), which focus
// on problem-solving rather than algorithms, making them a great resource for
// developing computational thinking and getting familiar with a language. These
// problems also offer an accompanying question after the first one is solved, forcing
// students to iterate on their design to accommodate to the requirements.
//
// The class should be structured into three sections: independent work time,
// collaborative thinking, and lecture. Independent work time allow students to
// practice their computational thinking, research skills, and their proficiency
// with the programming language. Collaborative thinking allows for students to
// review each other codes and explain their reasoning, allowing them to review their
// own reasoning. Lecture allow instructor to go over a preferred solution, either
// to highlight a language feature, a standard library to use, or an efficient manner
// to approach a genre of problems. Independent work should be $3/5$ of the class time,
// where collaborative thinking is encouraged to be $1/5$, however, lecture time can
// take more or all of collaborative thinking to better emphasize the reason for
// choosing the problem.
This section utilizes programming problems from _Advent of Code_
#cite(<advent>), focusing on
problem-solving rather than algorithms. These problems are an excellent resource for developing
computational thinking and familiarity with a new programming language. Additionally, they offer
accompanying questions that force students to iterate on their designs to meet requirements.
// The class structure consists of three sections: independent work time, collaborative thinking,
// and lecture. Independent work time allows students to practice computational thinking, research
// skills, and proficiency with the programming language. Collaborative thinking enables students to
// review each other's code and explain their reasoning, facilitating self-review. Lecture time
// allows the instructor to present preferred solutions, highlighting language features, recommended
// libraries, or efficient problem-solving approaches. Independent work should occupy $3/5$ of the
// class time, while collaborative thinking should be encouraged for $1/5$ of class time.
// However, lecture time may extend into collaborative thinking to emphasize why
// the problem was chosen. The amount of time for this section of the course is dependent
// on how efficient the students get with the language, but a maximum of 3 weeks.
The class structure comprises three sections: independent work time, collaborative thinking, and
lecture. During independent work time, students practice computational thinking, research skills,
and proficiency with the programming language. Collaborative thinking allows students to review
each other's code and explain their reasoning, fostering self-review. Lecture time is reserved
for the instructor to present preferred solutions, emphasizing language features, recommended
libraries, or efficient problem-solving approaches.
Independent work should consume $3/5$ of class
time, with collaborative thinking encouraged for $1/5$. However, lecture time may overlap with
collaborative thinking to emphasize why the problem was selected.
The duration of this course section is
dependent on students' language proficiency, maximum 2 weeks.
#figure(
rect(```
package aoc
import "core:strings"
...
bad_words :: []string{"ab", "cd", "pq", "xy"}
part_1 :: proc(file: ^string) -> (total: int) {
line_loop:
for line in strings.split_lines_iterator(file){
for word in bad_words {
if strings.contains(line, word) {
continue line_loop
}
}
...
```),
caption: [A snippet of _Odin_ code solving _Advent of Code_ problem: Day 5 of
2015#footnote[https://adventofcode.com/2015/day/5]],
)<advent_code>
== Learning Raylib with Conway's Game of Life: 4 Weeks
// Students will be learning Raylib through the making of a simple game. This will
// allow them to experiment with rendering to the screen, capturing user input, and
// UI implementation. _Conway's Game of Life_ allows students to focus on continually
// adding new features, rather than trying to figure out how to make the game. In doing
// so students are given the freedom to explore _Raylib_, and make the game their
// own, adding a personal connection to the project.
Students will learn _Raylib_ by creating a simple game, such as _Conway's Game of Life_. This
approach enables them to experiment with rendering to the screen, capturing user input, and
implementing UI elements. By focusing on continually adding new features to the game rather than
figuring out how to make it, students have the freedom to explore _Raylib_ and personalize their
project. This fosters a personal connection to the game and enhances their understanding of
_Raylib_'s capabilities.
// Classes are simply used for working on the project. Students are encouraged to come
// to class to share their ideas, show off their game, and/or just have a dedicated
// work space. The game does have requirements, but they should be vague to allow for
// student's creativity. For _Game of Life_ the requirements would be: control size of
// cells and window, save states for player's creations, a UI, and game speed control.
// Depending on the students' programming proficiency, the instructor may want to
// add or remove requirements to better optimize the difficulty to promote learning.
// Programming time also gives free time to the instructor to program their own hobby project,
// catch up on work, or complete the same project the students are.
Classes serve as dedicated workspaces for students to collaborate on their project.
They are encouraged to attend to share ideas, showcase their progress
or simply
work on their game. While the project has requirements, they are intentionally
vague to foster student creativity. For example, in the case of the _Game of Life_
project, requirements might include controlling the size of cells and the window,
implementing save states for player creations, adding a UI, and providing game speed
control. Depending on students' programming proficiency, the instructor may adjust
requirements to optimize difficulty to promote learning. Additionally, programming
time allows the instructor to have free time to pursue their own hobby projects,
catch up on work, or work on the same project alongside students.
// #figure(
// rect(```
// package game_of_life
// import rl "vendor:raylib"
// ...
// draw_logic_game_of_life :: proc(curr_cell: cell,
// x, y: int, game: ^Game) {
//
// if curr_cell.alive {
// rl.DrawRectangle(
// i32(x * int(game.cell_size)),
// i32(y * int(game.cell_size)),
// i32(game.cell_size),
// i32(game.cell_size),
// game.colors.live_cells,
// )
// }
// }
// ...
// main :: proc() {
// ...
// rl.InitWindow(
// game.window_size,
// game.window_size,
// "Game of Life"
// )
// defer rl.CloseWindow()
// rl.SetTargetFPS(60)
// game_init(&game)
//
// for !rl.WindowShouldClose() {
// player_control(&game)
// if game_speed(&game) {
// game_play(&game)
// }
// draw_game(&game)
// }
// }
// ```),
// caption: [Another snippet of _Odin_ code, but utilizing _Raylib_ to create an early
// implementation of _Conway's Game of Life_]
// )<odin_raylib>
#figure(
rect(```
package game_of_life
import rl "vendor/raylib"
...
game_draw :: proc(size: int) {
rl.BeginDrawing()
defer rl.EndDrawing()
rl.ClearBackground(rl.YELLOW)
for &row, y in game_space {
if y >= size {
break
}
for &space, x in row {
if x >= size {
break
} else if space {
rl.DrawRectangle(
i32(x * cell.width),
i32(y * cell.height),
i32(cell.width),
i32(cell.height),
rl.PINK,
)
}
}
}
}
...
main :: proc() {
size := SMALL
cellsize := game_creator(size)
game_init(cellsize, cellsize, size)
rl.InitWindow(i32(size * cell.width), i32(size * cell.height), "Game of Life")
defer rl.CloseWindow()
rl.SetTargetFPS(30)
for !rl.WindowShouldClose() {
game_draw(size)
game_play(size)
}
}
```),
caption: [Another snippet of _Odin_ code, but utilizing _Raylib_ to create an early
implementation of _Conway's Game of Life_]
)<odin_raylib>
// There are two parts to this section, first and second iteration. The first iteration
// gives students two weeks to implement _Game of Life_. To which afterward they let
// other students to read their code and give feedback #footnote[Instructors can choose their
// preferred choice of code hosting. Whatever makes it easier to review and share code with
// students and the instructor.]. With that feedback they undergo their second iteration
// to which will be the final product and what they'll officially be graded on; not only
// by the instructor, but also their peers.
This section consists of two parts: the first and second iterations. During the
first iteration, students have two weeks to implement the Game of Life.
Afterwards, students read their peer's code and provide feedback.
// #footnote[Instructors can choose their preferred code hosting platform for this purpose.].
With this feedback, students undergo their second iteration, which will serve as the final
product graded by both the instructor and their peers.
== Group Project and Code Reviews: 8 Weeks
// This section of the course utilizes the structure of Ousterhout's course, but with
// an emphasis on enabling student's creativity with programming, rather than
// software design. This is where this section then takes inspiration from game jams,
// as students are not given a requirement, but just a theme/mechanic/genre/application
// to incorporate in their project. Giving them a main goal, but allowing them to
// explore, experiment, and iterate on their proficiency with the language, knowledge
// of the library, computational thinking skills, and build their confidence.
This section of the course adopts the structure of Ousterhout's course, with a focus
on fostering students' creativity in programming rather than software design.
Drawing inspiration from game jams, students are provided with a theme, mechanic,
genre, or application to incorporate into their project. This approach gives them
a main goal while allowing for exploration, experimentation, and iteration. It
serves as an opportunity for students to enhance their proficiency with the language,
deepen their knowledge of the library, develop computational thinking skills, and
build confidence in their abilities.
// Students are given four weeks to develop their initial concept, thus a finished level
// to utilize the core program feature is a requirement for the first code review.
// The next four weeks require students to then iterate on their program based on the
// feedback, similar to the previous project. However, students are now in pairs, allowing
// them to tackle more complex projects or develop a better level of polish on their
// program. The projects should then take around 1000 - 1500 lines of code per student
// #cite(<john_programming>),
// this is not a concrete number, as students may need fewer lines of code to express
// their idea in its full intent.
Students have four weeks to develop their initial concept, aiming to produce a
finished level that utilizes the core program feature for the first code review.
Subsequently, the following four weeks are dedicated to iterating on their program
based on feedback, similar to the previous project. However, students are now paired
up, allowing them to tackle more complex projects or achieve a higher level of
polish. Each project should ideally consist of around 1000 - 1500 lines of code
per student#cite(<john_programming>), although this is not a strict requirement as
the number may vary depending on how efficiently students express their ideas.
// Much of this section does the same as the previous one, where students are just given
// dedicated time to work and explore. Instructors free time to use for their own
// benefit. The only major difference is giving students more time to create a more complex
// system than the previous one.
This section largely mirrors the previous one, providing students with dedicated time
to work and explore while giving instructors flexibility for personal pursuits.
The main distinction lies in affording students more time to develop a more complex
system compared to the previous project.
== Drawbacks of the Course
// The largest drawback of the course is the amount of reading the instructor must do.
// This limits the scalability of course, and though the first section can be automated
// easily, the core of the course are the projects. Small classes are the only manner to
// conduct this course, as the instructor does not want to overload themselves to
// review so much code.
The primary drawback of the course is the substantial amount of reading required
by the instructor, which limits its scalability#cite(<john_programming>).
While the first section can be
automated relatively easily, the heart of the course lies in the projects. Therefore,
small class sizes are necessary to conduct the course effectively, as the instructor
aims to avoid overwhelming themselves with the task of reviewing large volumes of code.
// The utilization of competitive programming is more for the problems utilized
// in them. These problems are open-ended allowing for students to creative apply
// their problem-solving skills and previous knowledge, utilizing computational thinking.
// The difficulty of these problems can be tuned to the skill level of the
// students. Competitive programming has a focus on algorithms, allowing for students
// to review their knowledge on algorithms, and strengthening their pattern recognition,
// understanding of, and applicative use of algorithms. By utilizing these strengths
// from competitive programming, one can teach new programming languages more efficiently.
// As students will be hands-on with the language, and reapplying previous knowledge
// and skills from previous language(s), allowing for faster pickup. Competitive
// programming offer students an environment to gauge and improve their skills and
// knowledge of fundamental computer science, allowing for development of
// computational thinking and an efficient manner to teach new languages.
// The use of competitive programming primarily revolves around the problems it
// presents. These problems are typically open-ended, allowing students to creatively
// apply their problem-solving skills and leverage their computational thinking abilities.
// The difficulty level of these problems can be adjusted to accommodate students' skill
// levels. Competitive programming focuses on algorithms, providing students with
// opportunities to reinforce their understanding and application of algorithms, as
// well as enhance their pattern recognition skills. By harnessing these aspects of
// competitive programming, one can effectively teach new programming languages.
// Students engage hands-on with the language, drawing from their previous knowledge
// and skills, which facilitates faster learning. Competitive programming environments
// allow students to assess and enhance their fundamental computer science knowledge,
// fostering the development of computational thinking and providing an efficient method
// for learning new languages.
//
// // Hackathons are like marathons, each person have their own goal in mind. However,
// // the most important part is finishing, allowing the participant to measure their
// // ability and challenge themselves in a timeframe to either learn something new,
// // or to see how far they've come, or compare themselves to others. By attending these
// // events participants are also able to meet others and share ideas in a safe place,
// // as the focus of the hackathon can be used to facilitate conversations or introduce
// // participants to new technologies and different sectors of the industry. By utilizing
// // the structure of hackathons, course design can instill intrinsic reasons for
// // participants to keep learning/practice the technology or as stepping stones to
// // a different sector they did not know about. Hackathons focus on a specific technology
// // or idea, allowing a main goal in mind, but also open-ended to where participants can
// // create their own goals to further motivate them in their future careers or hobbies.
//
// // Rewrite to include notes points.
// Hackathons resemble marathons in that each participant has their own individual
// objectives.#cite(<kris_hackathons>) However, the primary emphasis lies in completing the challenge within
// // I want to change this part. I feel I can expand on it more, allowing game
// // jams to take a "smaller role"
// a specified timeframe, enabling participants to gauge their abilities, challenge
// themselves, and potentially learn something new. Attending these events also provides
// an opportunity for participants to connect with others, share ideas, and explore
// new technologies and industry sectors in a supportive environment. By leveraging
// the structure of hackathons, course design can instill intrinsic motivations for
// participants to continue learning or practicing a particular technology, or to
// explore new career opportunities. Hackathons typically focus on a specific technology
// or concept, providing a clear goal while also allowing participants the freedom
// to set their own objectives, thereby further motivating them in their future endeavors.
//
// // Game jams and hackathons are similar, but differ in the environment they foster;
// // as game jams take on a play environment, allowing participants to focus on building
// // their project, rather than being distracted by competition. Competition is a
// // good motivator, but by allowing participants to focus on building their project,
// // they are able to explore new manners to creative solve problems or come up
// // with a new novel way; whereas competition can develop a META, stifling creativity.
// // Game jams can also be done with preexisting projects, allowing for more complex
// // projects to arise. Lastly, game jams offer a collaborative space for feedback
// // and review from other participants, encouraging the development of intrapersonal
// // and social skills.
//
// While game jams and hackathons share similarities, they differ in the environments
// they cultivate. Game jams prioritize a playful atmosphere, enabling participants
// to concentrate on project development without the distraction of competition#cite(<game_jams>).
// While competition can serve as a motivator, the absence of it in game jams allows
// participants to explore innovative problem-solving approaches and novel ideas without
// the constraints of a competitive mindset, which can sometimes stifle creativity.
// Additionally, game jams accommodate preexisting projects, facilitating the emergence
// of more intricate and advanced creations#cite(<blow_depth_jam>). Moreover, game jams provide a collaborative
// platform for feedback and peer review, fostering the growth of interpersonal and
// social skills among participants.
//
// // Conclusion
// // By utilizing the design of these programming activities, a course can be developed
// // that enables the practice of fundamental computer science concepts and the
// // exercising of computational thinking. Allowing students to gain a more intrinsic
// // appreciation of the discipline and being able to incorporate their own ideas
// // in their projects.
//
// By leveraging the design of these programming activities, a course can be crafted
// to facilitate the practice of fundamental computer science concepts and the cultivation
// of computational thinking skills. This approach empowers students to develop a
// deeper intrinsic understanding of the discipline while fostering the ability to
// integrate their own ideas into their projects.
//
// == Week 1-3: Learning Odin Through Advent of Code
//
// // To enable the focus on computational thinking and empowering students, tools must
// // be chosen carefully. Though tools can make it easier to develop on certain platforms,
// // or to better use certain libraries, to promote computational thinking the environment
// // must be open. Allowing students to explore different tools to enable their development
// // with the tools you force them to use. These tools can be programming languages,
// // libraries, or developer environments; to which influences the different domains
// // that students are exposed to, due to the abstraction they will commonly be
// // working in. Thus, by stripping the complexity and needed tools to complete
// // assignments allows for focus on the concepts being taught in the course.
//
// To foster computational thinking and empower students, tool selection is crucial.
// While certain tools may facilitate development on specific platforms or enhance
// utilization of particular libraries, it's essential to maintain an open environment.
// This enables students to explore various tools and develop proficiency based on
// their preferences and needs. Tools encompass programming languages, libraries,
// and developer environments, influencing the domains students encounter due to
// the abstractions they commonly work with. Therefore, simplifying complexity and
// limiting required tools for assignments allows for focused learning on the core
// concepts of the course.
//
// // The first three weeks is the introduction to one of the two core tools for the
// // course. Utilizing competitive programming problems will accelerate the learning
// // of the tool: a programming language. This does not necessarily have to teach a new
// // language, but can also be a review of a language and on core algorithms the
// // instructor might emphasize by the projects they may choose. In doing so, allows
// // students to gauge their skills and gives instructor's information on the skill
// // level of the students; allowing for instructors to dynamically alter the course
// // to fit a difficulty on the student.
//
// During the initial three weeks, students are introduced to one of the two core
// tools for the course. Utilizing competitive programming problems accelerates the
// learning process of this tool: a programming language. This approach not
// only facilitates the acquisition of new languages but also serves as a review for
// previously learned languages, emphasizing core algorithms through selected problems.
// By doing so, students can assess their skills, while instructors gain insights into
// their proficiency levels. This information allows instructors to dynamically adjust the
// course difficulty to suit the needs of individual students.
//
// // To allow for an open, dynamic environment, the activities will utilize _Advent of Code_
// // to teach the language. _Advent_ is different from other competitive programming
// // platforms for the openness of their input and outputs, as they give a unique
// // test case in a file and require a single output from processing such data. As well,
// // these same problems have a second additional question that require the participant
// // to rework their program to adapt to additional requirements. Requiring them to
// // learn their system and iterate on their program, rather than just trying to get
// // the right solution. Thus, if a participant had a flexible design on the first problem,
// // in theory they are able to complete the second problem faster; compared to poor
// // implementations that require a complete rewrite to fit the second problem requirements.
// // This open platform allow for participants to use the libraries in their language
// // to interact with their OS and files, forcing them to learn better parsing techniques
// // of files and strings. Overall, _Advent of Code_ allows deeper learning of a language
// // as the openness encourages the use of fundamental libraries/techniques in a language,
// // forces iteration on design (computational thinking) for the second question, and
// // still offers the benefits of competitive programming.
// === Why Advent of Code?
// To foster an open and dynamic learning environment, activities will utilize
// _Advent of Code_#cite(<advent>) to teach programming languages. _Advent of Code_ stands out from
// other competitive programming platforms due to its unique approach of providing
// input files with random test cases and a single output, along with an additional question
// that require participants to adapt and iterate on their programs. This format encourages
// participants to develop flexible designs and iterate on their solutions, enhancing
// their computational thinking skills.
// Overall, _Advent of Code_ promotes deeper learning of programming languages by emphasizing
// the utilization of core language features, fostering iterative problem-solving,
// and providing the benefits of competitive programming.
//
// === Why _Odin_?
// // Any language could have been chosen, it just has to enable the concepts or skills
// // the course requires. This course develops computational thinking, thus enabling
// // students to focus on their problem rather than learning the system around the language
// // to work on the problem is important. There are many popular simple languages
// // that can achieve this: Go, Python, JavaScript/TypeScript, C, etc.
// //
// // What _Odin_ offers is a battery included experience, a stepping stone to system
// // programming with a familiar face. The syntax is heavily influenced by Go and Python,
// // focusing on simplicity and the ability to do things in one manner, forcing one to
// // get it done, rather than being clever with the language and being simple to pick up.
// // This familiarity also encourages the idea that languages are tools, and the right
// // tool should be used for the right job. _Odin_ serves as a stepping stone to systems
// // due to its manual memory management and its close relation to C. Being able to
// // import C headers directly, and with its ideology on simplicity, _Odin_ is a trimmed
// // version of C, allowing the same power and flexibility, but reducing the number
// // of cuts from common mistakes. Lastly, _Odin_ is included with libraries able to
// // interact with the system's graphics and audio. Allowing for a standard environment
// // to start creating applications from the get-go, empowering new programmers to just start
// // creating. Overall, _Odin_ creates a standard environment that encourages play
// // by its simple rules, prepackaged libraries, and its ability to interact with the
// // most tenured programming ecosystem.
// Any language could have been chosen for this course as long as it enables the
// development of the required concepts and skills. The focus here is on computational
// thinking, allowing students to concentrate on problem-solving rather than getting
// bogged down in language intricacies. Simple languages like _Go_, _Python_, _JavaScript/
// TypeScript_, and _C_ are well-suited for this purpose.
//
// _Odin_#cite(<odin>) stands out by offering a comprehensive experience, serving as a gateway to
// system programming while maintaining a user-friendly interface. Its syntax, heavily
// influenced by _Go_ and _Python_, prioritizes simplicity and a straightforward approach
// to tasks, encouraging efficient problem-solving methods. This syntax similarity reinforces
// the idea that languages are tools, each suited for specific tasks. _Odin_'s manual
// memory management and close relationship with _C_ make it an ideal stepping stone
// to systems programming. Its ability to directly import _C_ headers and emphasis on
// simplicity make it a streamlined version of _C_, retaining its power and flexibility
// while reducing common mistakes.
//
// Moreover, _Odin_ comes with libraries for system graphics, networking and audio interaction,
// providing a standardized environment for creating applications right from the start.
// This empowers new programmers to dive into coding without unnecessary complexities.
// Overall, _Odin_ fosters a conducive environment for experimentation, thanks to its
// straightforward syntax, bundled libraries, and seamless integration with an established
// programming ecosystem.
//
// === Structure of Class
// // Utilizing _Advent of Code_ and _Odin_, class can be divided into three sections:
// // independent problem-solving, collaborative thinking, then a walkthrough of a
// // preferred solution. Depending on the problem, the instructor can dynamically allot
// // time for each section. Independent problem-solving involves independent research skills
// // as well, as students will need to look through documentation or instructor
// // supplemented material to better aide them with the problem. Allowing the students
// // to also familiarize themselves with the content before the instructor goes over it.
// // Collaborative thinking allows for students to either help each other or
// // brainstorm methods to tackle the problem. The walkthrough is the instructor's
// // time to teach, allowing them to either go through the whole problem or specific
// // sections to emphasize the concept they want to instill on the students.
// //
// // Independent and collaborative time gives the instructor the free time. Either by
// // demonstrating their programming skills on the board, either with the same language
// // or one they've been meaning to try for a while. Allows them to prepare how they may
// // want to teach the solution, as they can overhear the students as they brainstorm
// // together or if they want to walk the classroom to see how the students are doing.
// // The efficient use of this free time is key to continually develop the course through
// // the semester and to adapt the difficulty to better suit the student, as you should
// // not make it hard for hard sake.
// Class sessions can be structured into three
// segments: independent problem-solving, collaborative thinking, and instructor-led
// walkthroughs of solutions. Depending on the complexity of the problem, the instructor
// can dynamically allocate time for each section. Independent problem-solving tasks
// students with conducting research and exploring documentation or supplementary
// materials provided by the instructor, allowing them to familiarize themselves with
// the content before the instructor's explanation. Collaborative thinking encourages
// students to assist each other and brainstorm different approaches to tackle the
// problem collectively. The walkthrough session provides instructors with an opportunity
// to teach and emphasize key concepts by either reviewing the entire problem or focusing
// on specific sections.
//
// The independent and collaborative segments afford instructors valuable free time,
// which can be utilized for demonstrating programming skills on the board using familiar
// or new languages. This time allows instructors to prepare their approach to teaching
// the solution, observe student interactions, or monitor progress in the classroom.
// Efficient use of this free time is essential for continual course development and
// adapting the difficulty level to better suit students' needs without introducing
// unnecessary complexity.
//
// == Week 4-6: Learning Raylib - Game of Life
// // Once the students have a feel of the language, it is time to teach the library
// // that allows them to do more. Allowing the students to visually interact with their
// // programs builds their confidence in their programming skills and unveils the
// // perceived entry difficulty of creating applications or games. This can
// // inspire them to create more complex system as they feel empowered to do more.
// // Thus, choosing a library has the same requirements as the choosing the language.
// Once students are familiar with the language, it's time to introduce the library
// that expands their capabilities. Visual interaction with their programs boosts
// students' confidence in their programming skills and diminishes the perceived
// difficulty of creating applications or games. This empowerment often inspires
// students to tackle more complex systems. Therefore, selecting a library entails
// similar considerations as choosing the language itself.
//
// === Why Raylib?
// // _Raylib_#cite(<raylib>)was originally made as an educational tool to allow art
// // students to understand the basics of game programming#cite(<tj_raylib>). With the
// // ideology of how can one put pictures on the screen as simple as possible, it allows
// // for students to focus on their main objective, rather than dealing with the
// // another graphics as another hurdle. Due to it being made with _C99_ and utilizing
// // _OpenGL_, it is portable to different OSs/platforms, and to any language that supports
// // the _C_ FFI. There are bindings made for most of the popular languages, making it a
// // useful library to learn in general.
// _Raylib_#cite(<raylib>) was originally developed as an educational tool to help art students grasp
// the fundamentals of game programming#cite(<tj_raylib>). Created with the ideology of simplifying the
// process of displaying images on the screen, it allows students to focus on their
// primary objectives without being burdened by complex graphics implementation. Built
// using _C99_ and leveraging _OpenGL_, _Raylib_ is portable across various operating
// systems and platforms. Additionally, its compatibility with the _C_ Foreign Function
// Interface (FFI) enables integration with languages that support _C_. Thanks to
// bindings available for many popular languages, _Raylib_ serves as a valuable library
// for learning across different programming ecosystems and as a useful tool for future
// projects or hobbies.
//
// === Why Conway's Game of Life?
// // In truth, any sort of project could be used here. As long as you considered the
// // optimal difficulty for the students. Game of life is simple to implement and allows
// // the students to focus on the design of the code, rather than implementing it.
// // It also gives them more time to get used to the language, as it just uses basic
// // functions from _Raylib_. Which then allows them to focus on their software
// // design, to which allows them to come up with more creative and novel ways to
// // better implement the project.
// In truth, any sort of project could be used here, as long as you consider the
// optimal difficulty for the students. _Conway's Game of Life_, for instance, is simple
// to implement and allows the students to focus on the design of the code rather
// than its implementation. It also provides them with more time to become accustomed
// to the language, as it only utilizes basic functions from _Raylib_. This, in turn,
// enables them to focus on their software design, empowering them to devise more
// creative and novel ways to implement the project effectively.
//
// === Structure of the Three Weeks
// // This project has three checkpoints (one per week), to which each checkpoint introduces an additional
// // feature needing to be added to the project. Thus, the first week can be used for
// // simple rendering to the screen, then the next could be player input, a graphical
// // interface, sounds, loading assets, 3D, camera movement, etc. Instructors should then
// // also consider how this project can influence the difficulty of the next project.
// // Lastly, the project should enable the use of the desired feature, rather than
// // introducing it just because. Each additional feature should be purposeful, as it
// // is to inspire the students to do more, thus facilitating learning.
// //
// // This project can then be conceptualized as a hackathon, giving students a week
// // to implement the feature that is wanted. Each class is dedicated to students
// // working independently. This time can then be used as free time for the instructor,
// // preferably completing the project with the students, allowing the instructor to
// // gain a refresher of common pitfalls with the project, but also a better understanding
// // of how one can implement the project.
// //
// // Doing so with _Conway's Game of Life_, the first week is for implementation with
// // additional requirements, such as basic in-code customizability of game size, speed,
// // and window size, as well as an ability to pause. The second week entails
// // creating a graphical interface for the game, allowing for a player to change settings
// // with their mouse. The last week challenges the student's design as they need to
// // implement a new game mode. Rather than cells, they are _Pokémons_ that auto battle
// // each other base on their type#cite(<pokemon_game>). In doing so, they need to redesign
// // their UI, API, and maybe the manner they render to the screen. Creating a versatile
// // challenge to their computational thinking as they manage the system they created in
// // iterative spurts.
// This project is structured around three checkpoints, one for each week, with
// each checkpoint introducing an additional feature to be added to the project.
// Therefore, the first week can focus on simple rendering to the screen, while
// subsequent weeks could cover player input, graphical interfaces, sound implementation,
// asset loading, 3D elements, camera movement, and more. Instructors should also
// consider how this project's difficulty level can impact the next project in the
// curriculum. Moreover, it's essential that each feature added serves a purpose and
// enables the students to explore new concepts, rather than being included arbitrarily.
// Purposeful additions inspire students to push themselves further, thereby facilitating
// deeper learning.
//
// This project can be likened to a hackathon, where students are given a week to
// implement the desired feature independently. Class time is dedicated to students
// working on their projects autonomously. During this time, the instructor can also
// participate in the project, providing guidance and gaining insights into common
// challenges and implementation strategies.
//
// Using _Conway's Game of Life_ as an example, the first week focuses on implementing
// core functionality with additional requirements such as basic in-code customizability
// of game size, speed, and window size, along with the ability to pause. In the second
// week, students tackle the creation of a graphical interface, enabling players to adjust
// settings using their mouse. The final week presents a design challenge as students
// are tasked with implementing a new game mode where _Pokémons_ engage in auto battles
// based on their type#cite(<pokemon_game>). This requires a redesign of the UI, API,
// and potentially the rendering approach, providing a multifaceted challenge to
// students' computational thinking as they iterate on their project.
//
// == Week 7-14: Individual/Group Projects and Peer Reviews
// // Week 7-14 is up to the instructor to decide how to section. Depending on the project
// // complexity decides how much time the students need. A good criterion
// // is about 1000 lines of code per student in a three-week period#cite(<john_programming>).
// // However, instead of giving students a new feature implementation each week, you treat
// // the project time as a game jam; giving a theme or application genre. To which at the
// // end of the project time students share their projects with students to not only
// // interact with their final product, but also review their code, receiving suggestions or
// // showing off how they tackled their problems. Since everyone has skin in the game,
// // then criticism is a lot more constructive rather than subjective#cite(<blow_depth_jam>).
//
// == Drawbacks From This Design
// // The biggest drawback to this course design is the amount of code the instructor has to
// // read. Though reading this code does provide benefits for the
// // instructor#cite(<john_programming>), it requires a smaller class size. Though the
// // first section of the course can be done with a larger class, due to automated
// // checking. This barrier can be supplemented by using TAs, but could affect
// // grading quality. LLMs currently have a large enough token limit to analyze these
// // project sizes, allowing for faster analysis of code but with the chance of
// // hallucinations. _Gemini Pro_ alleviates this problem with the ability to refer
// // to inputted documents/files, however, it is currently slow and tests on how the
// // AI will affect the grading quality will need to be conducted.
// //
// // The students may also find the course as work heavy, depending on the project and
// // their ability. Though, the majority of class time is work time for the students.
// // Due to the nature of the course being project-based stress students more than a
// // traditional learning environment, as it may be a culture shock of learning method
// // but also the amount of coding one has to do.
// //
// // So, we are just going to copy John's course here. I think it does a
// // great job of what Blow had in mind what the Depth Jam was too.
// The primary challenge with this course design is the substantial workload it
// imposes on the instructor in terms of reviewing code. While code review offers
// valuable insights#cite(<john_programming>), managing larger class sizes may require
// automated checking, which could compromise grading quality. One potential solution
// is to employ teaching assistants (TAs), although this could introduce inconsistencies
// in grading. With advancements in language models (LLMs), such as the increased
// token limit, analyzing larger project sizes has become faster, albeit with the risk
// of hallucinations. Additionally, tools like _Gemini Pro_ offer the capability to
// reference inputted documents, although their current speed may hinder efficiency,
// and further testing is needed to evaluate their impact on grading quality.
//
// Moreover, students may perceive the course as excessively demanding, especially
// depending on the complexity of projects and their individual abilities. While the
// majority of class time is dedicated to project work, this project-based approach
// may induce more stress compared to traditional learning methods, potentially causing
// a culture shock for some students due to the intensive coding requirements.
//
= Conclusion
// I need to find the quote that says the students have to learn on their own.
// By utilizing game design concepts, courses can develop a play environment by correct
// tool and problem/environment choice. Doing
// so benefits both instructor and student. Students gain a deeper understanding
// of the curricula, but also are given more opportunities to apply fundamental computer
// science concepts (computational thinking). Utilizing activity-based learning to
// develop computational thinking and teach a programming language. Then by utilizing
// project-based learning to increase the complexity of the problems and giving students
// a manner to practice working with larger codebases, collaborate with others, and
// review code. Instructors benefit by being given free time to better improve the
// course, either by watching students, participating in the activities/projects, or
// trying out new tools and environments. This dynamic can be seen as a game developer
// and their players, to where the game developer want to instill the ideas they
// have onto the players, and players just want to have fun with the tools and
// environments/problems the developer gives them.
// By integrating game design principles into course development, educators can create
// a playful learning environment through thoughtful tool and problem/environment
// selection. This approach yields benefits for both instructors and students. Students
// not only gain a deeper comprehension of the curriculum but also have more opportunities
// to apply fundamental computer science concepts: computational thinking.
// Activity-based learning lays the foundation by fostering computational thinking and
// teaching programming languages, while project-based learning enhances complexity,
// enabling students to work with larger codebases, collaborate, and review code;
// better preparing them for future endeavors.
By incorporating game design principles into course development, educators can
craft a playful learning environment by carefully choosing tools and assignments.
This approach benefits both instructors and students. Students not only deepen
their understanding of the curriculum but also have increased opportunities to
apply fundamental computer science concepts and skills.
Activity-based learning establishes the groundwork by fostering computational
thinking through problem-solving and intentional tool practice, while project-based
learning increases difficulty and complexity, and integrates concepts into a cohesive system.
This allows students to work with larger codebases, collaborate, review code, and manipulate
systems, better preparing them for future endeavors in a playful learning
environment. Allowing students' ingenuity and mastery to be rewarded
through personal goals and desires.
Instructors also reap advantages by having free time to enhance the course. They
can observe students, actively participate in activities/projects, or experiment
with new tools and environments. This dynamic mirrors the relationship between game
developers and players: developers strive to impart their ideas to players, while
players seek enjoyment and engagement with the tools, environments, and problems
(games) made by developers.
|
https://github.com/typst/packages | https://raw.githubusercontent.com/typst/packages/main/packages/preview/songb/0.1.0/tests/autobreak.typ | typst | Apache License 2.0 | // SPDX-FileCopyrightText: 2024 <NAME> <<EMAIL>>
//
// SPDX-License-Identifier: CC0-1.0
#import "../autobreak.typ": autobreak;
#autobreak[
= Paragraph 1
]
#autobreak[
= Paragraph 2
should not break (enough space below Paragraph 1)
#lorem(500)
]
#autobreak[
= Paragraph 3
Paragraph 3 should break (not enough space below Paragraph 1+2)
#lorem(500)
]
#autobreak[
= Paragraph 4
Paragraph 4 should not break (can spread below paragraph 3 and next page)
#lorem(700)
]
#autobreak[
= Paragraph 5
Paragraph 5 should break (to prevent page turning)
#lorem(700)
]
#autobreak[
= Paragraph 6
Paragraph 6 should break (to prevent page turning)
#lorem(600)
]
#autobreak[
= Paragraph 7
Paragraph 7 should break twice? (not implement yet) (to prevent page turning)
#lorem(800)
]
|
https://github.com/typst/packages | https://raw.githubusercontent.com/typst/packages/main/packages/preview/grayness/0.1.0/example.typ | typst | Apache License 2.0 | #import "@local/grayness:0.1.0":*
#let data = read("Arturo_Nieto-Dorantes.webp",encoding: none)
#set page(height: 12cm, columns: 3)
Unmodified WebP-Image:
#show-image(data, width:90%)
Grayscale:
#grayscale-image(data, width:90%)
#colbreak()
Flip (vertically):
#flip-image-vertical(data,width:90%)
Transparency:
#transparent-image(data,width:95%)
#colbreak()
Blur:
#blur-image(data, sigma: 10, width:90%)
Crop:
#crop-image(data, 100, 120, start-x: 190, start-y: 95, width: 53%)
|
https://github.com/ericthomasca/resume | https://raw.githubusercontent.com/ericthomasca/resume/main/modules_en/skills.typ | typst | // Imports
#import "@preview/brilliant-cv:2.0.1": cvSection, cvSkill, hBar
#let metadata = toml("../metadata.toml")
#let cvSection = cvSection.with(metadata: metadata)
#cvSection("Skills")
#cvSkill(
type: [Languages],
info: [C\# #hBar() Python #hBar() Java #hBar() Go #hBar() Bash #hBar() JavaScript #hBar() TypeScript]
)
#cvSkill(
type: [Web],
info: [React #hBar() Next.js #hBar() Material-UI #hBar() ASP.NET #hBar() HTML5 #hBar() CSS3]
)
#cvSkill(
type: [Backend],
info: [Node.js #hBar() Express #hBar() SQL Server #hBar() MongoDB #hBar() PostgreSQL]
)
#cvSkill(
type: [DevOps & Cloud],
info: [AWS #hBar() Docker #hBar() Linux #hBar() Azure DevOps]
)
|
|
https://github.com/The-Notebookinator/notebookinator | https://raw.githubusercontent.com/The-Notebookinator/notebookinator/main/globals.typ | typst | The Unlicense | #let frontmatter-entries = state("frontmatter-entries", ())
#let entries = state("entries", ())
#let appendix-entries = state("appendix-entries", ())
#let glossary-entries = state("glossary-entries", ())
|
https://github.com/MultisampledNight/flow | https://raw.githubusercontent.com/MultisampledNight/flow/main/src/gfx/maybe-stub.typ | typst | MIT License | // Intends to reduce query times hugely.
// When not calling any cetz functions, only importing and parsing it takes a significant amount of
// time.
// Hence this module wraps cetz and imports it only if cfg.render=true.
// Otherwise it offers do-nothing stubs in place.
#import "../cfg.typ"
#let cetz = if cfg.render {
import "@preview/cetz:0.2.2"
cetz
} else {
import "stub.typ"
stub
}
|
https://github.com/protohaven/printed_materials | https://raw.githubusercontent.com/protohaven/printed_materials/main/class-handouts/class-wood_101-shop_basics.typ | typst |
#import "/meta-environment/env-protohaven-class_handouts.typ": *
#show: doc => class_handout(
title: "Basic Equipment Clearance",
category: "Wood",
number: "101",
clearances: ("Table Saw", "Band Saw (Wood)", "Drill Press (Wood)", "Sanding Table", "Miter Saw", "Spindle Sander", "Disc/Belt Sander"),
instructors: ("Someone",),
authors: ("<NAME> <<EMAIL>>",),
draft: true,
doc
)
// Content goes here
= Welcome
Welcome to the Woodshop Basic Equipment Clearance class at Protohaven!
#set heading(offset:1)
#include "/common-policy/shop_rules.typ"
#include "/common-policy/tool_status_tags.typ"
#include "/common-policy/filing_a_tool_report.typ"
#set heading(offset:0)
#pagebreak()
= Safety
// Important safety notes for this particular class
If you feel unsure of something, feel free to ask!
= Introduction
== Learning Objectives
== Terminology
= Tools
#set heading(offset:1)
// #include "/common-tools/TOOLNAME.typ"
#include "/common-tools/table_saw.typ"
#set heading(offset:0)
= Resources
// == Acknowledgments |
|
https://github.com/ilsubyeega/circuits-dalaby | https://raw.githubusercontent.com/ilsubyeega/circuits-dalaby/master/Type%201/2/46.typ | typst | #set enum(numbering: "(a)")
#import "@preview/cetz:0.2.2": *
#import "../common.typ": answer
2.46 다음 회로에서 $I$를 구하라. 이때 모든 저항의 단위는 $ohm$ 이다.
#answer[
Delta to Y를 사용한다.
2, 2, 4옴으로 이루어진 Delta를 Y로 변환한다.
위에서부터
$"sum" = 2+2+4 = 8$
$R_1 = (2 dot 2) / 8 = 0.5 ohm$
$R_2 = (2 dot 4) / 8 = 1 ohm$
$R_3 = (4 dot 2) / 8 = 1 ohm$
$therefore R_"eq" = 3, I = 12 / R_"eq" = 4 A$.
] |
|
https://github.com/CaldeCrack/Typst-Templates | https://raw.githubusercontent.com/CaldeCrack/Typst-Templates/main/README.md | markdown | # Typst-Templates
Typst personal templates for various purposes
## Templates
- [Default](template.typ): Creada basándose en la template de auxiliares de <NAME> [[fuente](https://latex.ppizarror.com/auxiliares)]
## Modules
- [Auto Rectangle](modules/auto_rect.typ): Creación más rápida de rectángulos con valor del parámetro centrado
- [Auto Table](modules/auto_table.typ): Creación más sencilla de tablas tan solo pasando los valores que se quieren agregar
|
|
https://github.com/Myriad-Dreamin/typst.ts | https://raw.githubusercontent.com/Myriad-Dreamin/typst.ts/main/fuzzers/corpora/layout/par-justify-cjk_01.typ | typst | Apache License 2.0 |
#import "/contrib/templates/std-tests/preset.typ": *
#show: test-page
// Japanese typography is more complex, make sure it is at least a bit sensible.
#set page(width: auto)
#set par(justify: true)
#set text(lang: "jp", font: ("Linux Libertine", "Noto Serif CJK JP"))
#rect(inset: 0pt, width: 80pt, fill: rgb("eee"))[
ウィキペディア(英: Wikipedia)は、世界中のボランティアの共同作業によって執筆及び作成されるフリーの多言語インターネット百科事典である。主に寄付に依って活動している非営利団体「ウィキメディア財団」が所有・運営している。
専門家によるオンライン百科事典プロジェクトNupedia(ヌーペディア)を前身として、2001年1月、ラリー・サンガーとジミー・ウェールズ(英: <NAME> "Jimbo" Wales)により英語でプロジェクトが開始された。
]
|
https://github.com/vsheg/intermediate-ml | https://raw.githubusercontent.com/vsheg/intermediate-ml/main/03-nonlinear/01-nonlinear-ls.typ | typst | #import "@preview/physica:0.9.3": *
#import "../defs.typ": *
#import "../template.typ": *
#show: template
= Non-linear Optimization: Newton --- Gauss Method
The Newton–Gauss method is a second-order optimization technique for quadratic functions,
utilizing a linear approximation of the optimized function at each step. It is applied to
solve nonlinear least squares problems, effectively reducing them to a sequence of linear
least squares problems.
== Gradient and Hessian of the Loss Function
Given the quadratic loss function
$
Q(xb) = sum_(xb in X^ell) (a(xb, tb) - y(xb))^2
$
we can express the gradient and Hessian of the function in terms of the model's
parameters:
#note[
gradient is the column vector:
$ nabla f(bold(x)) := vec(pdv(f(xb), x_1), dots.v, pdv(f(xb), x_k)), $
and $f'_j$ denotes $j$th component of the column
]
1. The gradient components are
$
Q'_j
&= pdv(Q, theta_j) \
&= 2 sum_(xb in X^ell) (a(xb, tb) - y(xb)) dot pdv(a(xb, tb), theta_j)
$
2. The Hessian components are
$
Q''_(i,j) &= pdv(Q, theta_i, theta_j) \
&= 2 sum_(xb in X^ell) pdv(a(xb, tb), theta_i) pdv(a(xb, tb), theta_j) - 2
sum_(xb in X^ell) (a(xb, tb) - y(xb)) dot pdv(a(xb,
tb), theta_i, theta_j).
$
== Linear Approximation of the Algorithm
Apply a Taylor series expansion of the algorithm up to the linear term near the current
approximation of the parameter vector $hat(tb)$:
$
a(xb, tb) = ub(a(xb, hat(tb)), const) + sum_j ub(pdv(a(xb, hat(tb)), theta_j), const_j)
ub((theta_j - hat(theta)_j), delta theta_j) + O(norm(tb - hat(tb))^2),
$
$a(xb, hat(tb))$ is constant, and the linear term is the sum of the partial derivatives of
$a(xb, hat(tb))$ with respect to the parameters $theta_j$. The higher-order terms are
negligible and will be omitted below.
Differentiate the linear approximation of the algorithm:
$
pdv(, theta_j) a(xb, tb)
&approx 0 + ub(pdv(a(xb, hat(tb)), theta_j), const_k) dot 1 + cancel(O(norm(tb - hat(tb))^2)) \
&= const_j
$
The components of the sum depending on $theta_(j!=k)$ was zeroed out in the
differentiation over $theta_k$.
Substitute the obtained derivative into the expression for the Hessian:
$
Q''_(i,j)
& approx 2 sum_(xb in X^ell) ub(pdv(a(xb, hat(tb)), theta_i), const_i)
ub(pdv(a(xb, hat(tb)), theta_j), const_j) - cancel(2 sum_(xb in
X^ell) (a(xb, tb) - y(xb)) dot 0)
$
The linear term will be zeroed out in the second differentiation and will not enter the
Hessian.
== Matrix Formulation of the Optimization Step
Introduce the matrix of first partial derivatives and the algorithm's response vector at
the current approximation of the parameters $hat(tb)$:
$
D := {pdv(a(xb_i, hat(tb)), theta_j)}_(i,j), quad bold(a) :=
vec(a(xb_1, hat(tb)), dots.v, a(xb_ell, hat(tb)))
$
matrix $D$ and vector $bold(a)$ depend on the point of expansion $hat(tb)$ and are
recalculated at each optimization step.
The gradient and Hessian (at each step) are calculated using the matrix $D$:
$
Q' = D^Tr (bold(a) - bold(y)), quad Q'' = D^Tr D (bold(a) - bold(y))
$
#note[Newton --- Rafson method is a second-order optimization technique that provides fast
convergence. Newton–Gauss method is an approximate second-order method that uses a linear
approximation of the optimized function at each step.]
The optimization step of the Newton --- Rafson method is also expressed in terms of the
matrix $D$:
$
tb<- tb - gamma dot overbrace(ub((D^Tr D)^(-1) D^Tr, D^+)
ub((bold(a) - bold(y)), bold(epsilon)), delta tb)
$
#note[The nonlinear optimization problem is reduced to a sequence of linear problems: at each
iteration, a linear expansion of the function is made, matrices are calculated, and a
(new) system of linear equations is solved.]
The optimization step vector at each iteration can be determined from the linear system in
any of these formulations:
$
ub(bold(epsilon), bold(y)) = D dot ub(delta tb, bold(beta))
quad <=> quad delta tb = D^+ bold(epsilon) quad <=> quad norm(D dot delta
tb - bold(epsilon))^2 -> min_bold(beta)
$
#note[The method is a second-order approximation method, providing fast convergence and slightly
inferior accuracy compared to the Newton–Raphson method.]
|
|
https://github.com/edgarogh/f4f1eb | https://raw.githubusercontent.com/edgarogh/f4f1eb/main/demo_long.typ | typst | #import "template.typ": *
#set page(numbering: "1")
#show: project.with(
title: [Anakin \ Skywalker],
from_details: [
Appt. x, \
Mos Espa, \
Tatooine \
<EMAIL> \ +999 xxxx xxx
],
to_details: [
Sheev Palpatine \
500 Republica, \
Ambassadorial Sector, Senate District, \
Galactic City, \ Coruscant
],
)
Dear Emperor,
You will _never_ guess what happened to me last week-end! #lorem(200)
#lorem(220)
#lorem(180) Hence the scratch on my helmet.
<NAME>
|
|
https://github.com/1939323749/typst-zuotiben | https://raw.githubusercontent.com/1939323749/typst-zuotiben/main/README.md | markdown | # 25竟成计组做题本
基于typst制作的25竟成计组选择题做题本
## 使用方法
```bash
typst compile main.typ 25竟成计组选择题做题本.pdf
```
|
|
https://github.com/michalrzak/muw-typst-template | https://raw.githubusercontent.com/michalrzak/muw-typst-template/main/template.typ | typst | // Template created after the requirements in https://ub.meduniwien.ac.at/fileadmin/content/OE/ub/dokumente/Leitfaden_Studierende_Hochschulschriften_MedUni_Wien.pdf
#let project(
type: "",
title_en: "",
title_de: "",
targeted_acadmic_degree: "",
study_program: "",
conducted_at: "",
supervisors: (),
your_name: "",
your_orcid: "",
date: "",
place: "",
acknowledgements: [],
motivation: [],
abstract_en: [],
abstract_de: [],
bibfile: "",
abbreviations: (),
skip_figure_list: false,
skip_table_list: false,
skip_formula_list: false,
body,
) = {
// Set the document's basic properties.
set document(author: your_name, title: title_en)
set page(
// set required margins accordign to style document
margin: (left: 30mm, right: 25mm, top: 25mm, bottom: 25mm),
number-align: center,
)
// set a sans-serif font with letter size 12
// note that according to https://github.com/typst/typst/discussions/2919 typst does not directly allow setting header scaling, but their defaults essentially match the MUW requirements of 14 (12 * 1.2) and 16 (12 * 1.4)
set text(font: "New Computer Modern Sans", lang: "en", size: 12pt)
show math.equation: set text(weight: 400)
// Set paragraph spacing.
show par: set block(above: 1.2em, below: 1.3em)
set heading(numbering: "1.1")
// the MUW recommends line spacing 1.5
// unfortunately, "line spacing 1.5" is a vary vague term and is not easy to set in typst
// the following setting is not entirely 1.5 but comes close enough
set par(leading: 0.8em)
// set the numbering of equations (and automatically tables) to be per chapter
// reset figure and math equation counters with each heading
show heading.where(level:1): it => {
counter(figure.where(kind: table)).update(0)
counter(figure.where(kind: image)).update(0)
counter(math.equation).update(0)
it
}
// As per 08.03.24 (version 0.10.0) this does not work
// however acccording to https://github.com/typst/typst/issues/1057
// this has been already implemented. Should be fixed with
// the next realease of typst.
set figure(numbering: it => {
[#counter(heading.where(level:1)).display()\.#it]
})
set math.equation(numbering: it => {
[(#counter(heading.where(level:1)).display()\.#it)]
})
// ------------------------------------
// Title page.
set page(numbering: none)
let c = navy
let type_style(content) = {
set text(c, 2.1em)
content
}
let title_style(content) = {
set text(c, 2em, weight: 700)
content
}
let bold_regular_style(content) = {
set text(c, 1.2em, weight: 600)
content
}
let regular_style(content) = {
set text(c, 1.2em)
content
}
let small_style(content) = {
set text(c)
content
}
align(left, image("logo.svg", width: 26%))
align(center)[#type_style(type)]
linebreak()
align(center)[
#title_style(title_en)
#linebreak()
#linebreak()
#title_style(title_de)
]
linebreak()
align(center)[
#bold_regular_style("zur Erlangung des akademischen Grades")
#linebreak()
#regular_style(targeted_acadmic_degree)
#linebreak()
#small_style("an der")
#linebreak()
#bold_regular_style("Medizinischen Universität Wien")
#linebreak()
#regular_style("Studiendrichtung: ") #bold_regular_style(study_program)
]
linebreak()
align(center)[
#bold_regular_style("ausgeführt am:")
#linebreak()
#bold_regular_style(conducted_at)
]
linebreak()
align(center)[
#bold_regular_style("unter der Anleitung von:")
]
for supervisor in supervisors {
align(center)[#regular_style(supervisor)]
}
linebreak()
align(center)[
#bold_regular_style("eingereicht von")
#linebreak()
#regular_style(your_name)
#linebreak()
#linebreak()
#regular_style("ORCID:")
#regular_style(your_orcid)
]
linebreak()
align(center)[
#regular_style(place), #regular_style(date)
]
pagebreak()
// ------------------------------------
// Declaration of honesty
set page(numbering: "i")
counter(page).update(1)
heading(numbering: none, "Declaration of honesty")
text("Ich erkläre ehrenwörtlich, dass ich die vorliegende Abschlussarbeit selbstständig und ohne fremde Hilfe verfasst habe, andere als die angegebenen Quellen nicht verwendet habe und die den benutzten Quellen wörtlich oder inhaltlich entnommenen Stellen als solche kenntlich gemacht habe.")
linebreak()
linebreak()
linebreak()
line(length: 100%)
text(place)
text(", am")
h(50%)
text("Unterschrift")
pagebreak()
// ------------------------------------
// Acknowledgements
if acknowledgements != []{
heading(outlined: false, numbering: none, text(0.85em)[Acknowledgements])
acknowledgements
pagebreak()
}
// ------------------------------------
// Motivation
if motivation != []{
heading(outlined: false, numbering: none, text(0.85em)[Motivation])
motivation
pagebreak()
}
// ------------------------------------
// Abstract page English.
set page(numbering: "1")
counter(page).update(1)
heading(outlined: false, numbering: none, text(0.85em)[Abstract])
abstract_en
v(1.618fr)
pagebreak()
// ------------------------------------
// Abstract page German.
heading(outlined: false, numbering: none, text(0.85em)[Zusammenfassung])
abstract_de
v(1.618fr)
pagebreak()
// ------------------------------------
// Table of contents.
outline(depth: 3, indent: true)
pagebreak()
// ------------------------------------
// Main body.
set par(justify: true)
set text(hyphenate: false)
body
// ------------------------------------
// Bibliography
pagebreak()
bibliography(bibfile)
pagebreak()
// Lists
set page(numbering: "i")
counter(page).update(1)
// ------------------------------------
// List of figures
if not skip_figure_list {
heading(numbering: none, [List of Figures])
outline(
title: "",
target: figure.where(kind: image),
)
pagebreak()
}
// ------------------------------------
// List of tables
if not skip_table_list {
heading(numbering: none, [List of Tables])
outline(
title: "",
target: figure.where(kind: table),
)
pagebreak()
}
// ------------------------------------
// List of formulas
if not skip_formula_list {
heading(numbering: none, [List of Formulas])
outline(
title: "",
target: math.equation,
)
pagebreak()
}
// ------------------------------------
// List of abbreviations
if abbreviations != () {
heading(numbering: none, "List of Abbreviations")
linebreak()
for key in abbreviations.keys().sorted() {
[/ #key: #abbreviations.at(key)]
}
pagebreak()
}
// ------------------------------------
// Appendix
heading(numbering: none, "Appendix")
} |
|
https://github.com/piepert/philodidaktik-hro-phf-ifp | https://raw.githubusercontent.com/piepert/philodidaktik-hro-phf-ifp/main/src/parts/ephid/ziele_und_aufgaben/kompetenzen.typ | typst | Other | #import "/src/template.typ": *
== #ix("Kompetenzen", "Kompetenz")
// #show table: it => align(center, block(align(left, block(it))))
#def("Kompetenz")[
Kompetenzen "sind die bei Individuen verfügbaren oder durch sie erlernbaren kognitiven Fähigkeiten, um bestimmte Probleme zu lösen, sowie die damit verbundenen motivationalen, volitionalen und sozialen Bereitschaften und Fähigkeiten, um die Problemlösungen in variablen Situationen erfolgreich und verantwortungsvoll nutzen zu können.“#en[@Weinert2001_Kompetenz[S. 27f]]
]
#ix("Kompetenzen", "Kompetenz") sind grundlegend für die Unterrichtsplanung. Unterschiedliche Schulformen haben unterschiedliche #ix("Kompetenzen", "Kompetenz") und müssen bei der Unterrichtsplanung berücksichtigt werden. Genauere Erklärungen und #ix("Kompetenzen", "Kompetenz") sind im Abschnitt zu den Rahmenplänen beschrieben. Die übergeordnete #ix("Kompetenz") bildet in der Klasse 7-10 die #ix("Handlungskompetenz") und in der #ix("Sekundarstufe II", "Oberstufe, gymnasial", "Sekundarstufe II") die #ix("Reflexionskompetenz").#ens[Vgl. @MBWKMV_RPGS[S. 4 ff]][Vgl. @MBWKMV1996_RP56[S. 5 ff]][Vgl. @MBWKMV2002_RP710[S. 3 ff]][Vgl. @MBWKMV2019_RP1112[S. 7 ff]]
#table(columns: (50%, 50%),
stroke: none,
strong[#ix("Fachübergreifend", "Kompetenzen, fachübergreifend"),\ Klasse 5-10],
strong[#ix("Fachspezifisch Philosophie", "Kompetenzen, fachspezifisch für Philosophie"),\ #ix("Sekundarstufe II", "Oberstufe, gymnasial")], [
- #ix("Sozialkompetenz")
- #ix("Selbstkompetenz")
- #ix("Methodenkompetenz")
- #ix("Sachkompetenz")
#strong[$=>$ #ix("Handlungskompetenz")]
], [
- #ix("Wahrnehmungs- und Deutungskompetenz", "Wahrnehmungskompetenz", "Deutungskompetenz")
- #ix("Argumentations- und Urteilskompetenz", "Argumentationskompetenz", "Urteilskompetenz")
- #ix("Darstellungskompetenz")
- #ix("Praktische Kompetenz", "Praktische Kompetenz", "Kompetenz, praktische")
#strong[$=>$ #ix("Reflexionskompetenz")]
]
)
#task(key: "kompetenzbegriff-weinert")[Kompetenz][
Definieren Sie den Kompetenzbegriff nach Weinert!
][
Kompetenzen sind kognitive Fähigkeiten, um bestimmte Probleme zu lösen, sowie die damit verbundenen motivationalen, volitionalen und sozialen Bereitschaften und Fähigkeiten, um die Problemlösungen in variablen Situationen erfolgreich und verantwortungsvoll nutzen zu können.
]
#task[Qualitätskriterien der PhiD][
#ix("<NAME>", "<NAME>") stellt 1798 in der "Anthropologie in pragmatischer Hinsicht" drei Gebote zur
Erlangung von Weisheit auf. Nennen und erläutern Sie diese knapp in Hinblick auf
Qualitätskriterien von Philosophieunterricht.
][
#ix("Kant", "<NAME>") stellt drei Maximen auf:// #en[Vgl. Kant, Immanuel: Kants gesammelte Schriften. Hrsg. von der königlich preussischen Akademie der Wissenschaften. Berlin 1900 ff. S. 201.] // en in Aufgaben geht immernoch nicht
+ selbst denken
+ sich an die Stelle des Anderen denken
+ mit sich selbst einstimmig zu denken
Das "selbst denken" ist der Grundsatz des "#ix("Philosophieren mit Kindern", "Philosophieren mit Kindern", "PmK", "PwC")" statt "#ix("Philosophie für Kinder", "Philosophie für Kinder", "PfK", "P4C")". Es geht darum, die Kinder zum Philosophieren zu bringen. Als Qualitätskriterium kann es wie folgt formuliert werden: Der Philosophieunterricht bringt die SuS dazu, selbständig und aktiv zu philosophieren.
Mit #ix("Kants", "<NAME>") "sich an die Stelle des Anderen denken" ist gemeint, über den eigenen Horizont hinausblicken und die Positionen anderer erfassen zu können. Der Philosophieunterricht muss die SuS dazu bringen, sich mit anderen Meinungen auseinandersetzen zu können.
Das letzte Gebot, das "mit sich selbst einstimmig zu denken" heißt im Bezug auf den Philosophieunterricht, die Kinder dazu anzuregen, sich aus einem diversen Bild eine eigene Meinung bilden zu können. Es geht darum, die Argumentationen und Perspektiven anderer einbeziehen zu können, diese abzuwägen und zu einem begründeten Urteil kommen zu können. Als Qualitätskriterium könnte man es so auslegen: Der Philosophieunterricht bringt die SuS dazu, eine Meinung fällen und diese begründen zu können.
] |
https://github.com/goshakowska/Typstdiff | https://raw.githubusercontent.com/goshakowska/Typstdiff/main/tests/test_working_types/bullet_list/bullet_list_updated.typ | typst | - updated item
- second item
|
|
https://github.com/HollowNumber/DDU-Rapport-Template | https://raw.githubusercontent.com/HollowNumber/DDU-Rapport-Template/main/src/chapters/ordliste.typ | typst | #import "@preview/glossarium:0.2.3": make-glossary, print-glossary, gls, glspl
#set page(columns: 1)
#heading("Ordliste", numbering: none)
#print-glossary((
(
key: "moscow-method",
short: "MoSCoW",
long: "MoSCoW metoden",
desc: [
MoSCoW metoden er en prioriteringsmetode, der bruges til at prioritere krav i et projekt.
Den er delt op i fire kategorier: *Must Have* --- disse krav er de krav, der er nødvendige for at projektet kan blive en succes. *Should Have* --- disse krav er de krav, der er vigtige for at projektet kan blive en succes. *Could Have* --- disse krav er de krav, der er ønskelige for at projektet kan blive en succes. *Won't Have* --- disse krav er de krav, der ikke er nødvendige for at projektet kan blive en succes.
]
),
(
key: "bartles-taksonomi",
short: "Bartle's taksonomi",
long: "Bartle's taksonomi af spiller typer",
desc:
[
Bartles taksonomi af spiller typer er en model, der beskriver fire forskellige typer af spillere. Disse fire spiller typer leder alle sammen efter noget forskelligt i de spil, de spiller. *Explorers* --- disse spillere er interesserede i at udforske spillets verden og finde nye ting. *Socializers* --- disse spillere er interesserede i at interagere med andre spillere. *Achievers* --- disse spillere er interesserede i at opnå mål i spillet. *Killers* --- disse spillere er interesserede i at konkurrere med andre spillere.
]
),
(
key: "mda-framework",
short: "MDA framework",
long: "Mechanics-Dynamics-Aesthetics framework",
desc: [
MDA frameworket er en model, der beskriver hvordan spil kan blive analyseret. Den er delt op i tre kategorier: *Mechanics* --- disse er de regler, der er i spillet. *Dynamics* --- disse er de handlinger, der kan udføres i spillet. *Aesthetics* --- disse er de følelser, der opstår hos spilleren, når de spiller spillet.
]
),
)) |
|
https://github.com/Myriad-Dreamin/typst.ts | https://raw.githubusercontent.com/Myriad-Dreamin/typst.ts/main/fuzzers/corpora/visualize/pattern-simple_01.typ | typst | Apache License 2.0 |
#import "/contrib/templates/std-tests/preset.typ": *
#show: test-page
#set page(width: auto, height: auto, margin: 0pt)
#let pat = pattern(size: (10pt, 10pt), {
place(line(stroke: 4pt, start: (0%, 0%), end: (100%, 100%)))
place(line(stroke: 4pt, start: (100%,0%), end: (200%, 100%)))
place(line(stroke: 4pt, start: (0%,100%), end: (100%, 200%)))
place(line(stroke: 4pt, start: (-100%,0%), end: (0%, 100%)))
place(line(stroke: 4pt, start: (0%,-100%), end: (100%, 0%)))
})
#rect(width: 50pt, height: 50pt, fill: pat)
|
https://github.com/stefanocoretta/lexa-dict | https://raw.githubusercontent.com/stefanocoretta/lexa-dict/main/main.typ | typst | #let dict(contents) = {
// define abbreviations
show "noun": [n.]
show "verb": [v.]
show "adjective": [adj.]
show "adverb": [adv.]
show "particle": [ptc.]
show "numeral": [num.]
// Typeset each entry
for (entry, value) in contents [
// lexeme
#let has_hom = value.at("homophone", default: false)
#strong()[#value.entry#if has_hom != false [#sub()[#value.homophone]]]
// phonetic/phonemic transcription
#text(font: "Fira Sans", size: 0.8em)[/#value.phon/]
// horizontal space between lexeme/phon and rest
#box(width: 0.5em)
//
// senses
#let senses_n = value.senses.len()
#let sense_i = 1
#for (i, y) in value.senses {
// just insert the sense without numeric idx
// if only one sense
if senses_n > 1 {
"• " + strong(str(sense_i) + ". ")
sense_i = sense_i + 1
}
// pos
text(fill: blue, style: "italic")[#value.part_of_speech]
//
// inflectional classes
let has_infl = value.at("inflectional_features", default: false)
if has_infl != false [
// define classes abbreviations and styling
#show "reme": it => smallcaps()[r]
#show "lapy": it => smallcaps()[l]
(#text(fill: maroon)[#value.inflectional_features.class])
]
y.definition + " "
}
]
}
#align(center)[
#text(size: 30pt, weight: "bold")[A Thale dictionary]
#text(size: 15pt, weight: "bold")[Stefano Coretta]
]
#v(40pt)
#show: doc => columns(2, doc)
#dict(
yaml("lexicon.yaml")
) |
|
https://github.com/takotori/PhAI-Spick | https://raw.githubusercontent.com/takotori/PhAI-Spick/main/sections/leistung.typ | typst | = Leistung $P$
#grid(
columns: (auto, auto, auto),
gutter: 5pt,
[*Mittlere Leistung:*], $ macron(P) = W_"AB" /(Delta t) = (Delta E)/(Delta t) $, [],
[*Momentane \ Leistung:*], $ (d W)/(d t) = arrow(F) dot arrow(v) $, [],
[*Wirkungsgrad:*], $ eta = W_2/W_1 = P_2/P_1 $, [$W_1 P_1$: aufgenommene Leistung bzw. Arbeit \
$W_2 P_2$: nutzbare Leistung bzw. Arbeit
],
[*Vortriebskraft:*], $ F = P/v $, [],
[*Reibungs-\ koeffizient:*], $ mu = P /(F_G v) $, [],
[*Steigleistung:*], $ (d h)/(d t) = P / (F_G + P/v cot(alpha)) $, []
)
*Leistung auf Schiefen Ebene in Abhängigkeit von $s$ und $h$:*
$
W=(h+mu_R sqrt(s^2-h^2 ))m g
$
|
|
https://github.com/rabotaem-incorporated/probability-theory-notes | https://raw.githubusercontent.com/rabotaem-incorporated/probability-theory-notes/master/sections/04-discrete-random-processes/04-random-walk.typ | typst | #import "../../utils/core.typ": *
== Случайные блуждания
#th[
Случайное блуждание в соседние точки на прямой возвртано тогда и только тогда, когда $p = 1/2$.
]
#proof[
Не будем рассматривать дурацкие ситуации, когда $p = 0$ или $p = 1$. Тогда все состояние сообщающиеся, поэтому достаточно рассмотреть одно конкретное состояние. Например $0$. $p_(0, 0) (n) = 0$ если $n$ --- нечетное. $p_(0, 0) (2n) = C_(2n)^n p^n (1 - p)^n$. Тогда
$
sum_(n = 1)^oo p_(0, 0) (n) = sum_(n = 1)^oo p_(0, 0) (2n) = sum_(n = 1)^oo C_(2n)^n (p (1 - p))^n.
$
Знаем $C_(2n)^n sim 4^n/(sqrt(pi n))$. Тогда
$
C_(2n)^n (p (1 - p))^n sim 1/sqrt(pi n) (4 p (1 - p))^n <= (4 p (1 - p))^n.
$
Тогда
- $4 p (1 - p) = 1$, если $p = 1/2$, и ряд $sim sum 1/sqrt(pi n)$ и расходится.
- $4 p (1 - p) < 1$, если $p != 1/2$, и ряд $sum (4p (1 - p))^n$ сходится.
]
#th[
Рассмотрим произвольное симметричное случайное блуждание по $ZZ$ (не обязательно в соседние точки). $xi_1$, $xi_2$, ... --- независимые одинаково распределенные симметричные случайные величины. $S_n = xi_1 + xi_2 + ... + xi_n$.
Пусть $E abs(xi_1) < +oo$. Тогда такое случайное блуждание возвратно.
]
#proof[
$p_(0, 0) (n) = P(S_n = 0)$. Будем проверять условие возвратности. Распишем производящую функцию
$
G(z) := sum_(n = -oo)^(oo) P (xi_1 = n) z^n.
$
Это ряд Лорана, и, вообще говоря, мы такие не исследовали, но пофиг. При суммировании независимых случайных величин производящие ряды все равно перемножаются, поэтому $G_(S_n) (z) = G^n (z)$.
Теперь следующая проблема: мы хотим узнать $P(S_n = 0)$, но $0$ мы подставить не можем (мы на $z$ делим). Поэтому пишем теорему Коши:
$
P(S_n = 0) = 1/(2pi i) integral_(abs(z) = 1) (G^n (z)) / z dif z.
$
И тогда нам нужен ряд
$
1/(2pi i) sum_(n = 0)^oo integral_(abs(z) = 1) (G^n (z))/z dif z
$
Обосновать почему законно переставлять сумму с интегралом мы не можем, потому что нет равномерной сходимости. Поэтому рассмотрим такой ряд $0 <= x < 1$:
$
sum_(n = 0)^(oo) P(S_n = 0) x^n =
1/(2pi i) sum_(n = 0)^oo integral_(abs(z) = 1) underbrace((G^n (z))/z, "по модулю" <= 1) x^n dif z newline(=^"Фубини")
1/(2pi i) integral_(abs(z) = 1) 1/z sum_(n = 0)^oo x^n G^n (z) dif z =
1/(2pi i) integral_(abs(z) = 1) (dif z)/(z (1 - x G(z))).
$
Знаем $E abs(xi_1) < +oo$, $sum_(n = -oo)^oo P(xi_1 = n) = 1$. Что мы знаем про $G$?
$
G' (z) = sum_(n = -oo)^oo n z^(n - 1) P(xi_1 = n), quad G' (1) = sum_(n = -oo)^oo n P(xi_1 = n) = 0.
$
Причем $G(1) = 1$, поэтому $G(z) = 1 + o(1 - z)$, и $G(e^(i t)) = 1 + o(1 - e^(i t)) = 1 + o(t)$ при $t --> 0$.
Подставляем, и оцениваем $o(t) <= c t$:
$
1/(2pi i) integral_(abs(z) = 1) (dif z)/(z (1 - x G(z))) =
1/(2pi) integral_0^(2pi) (dif t)/(1 - x G(e^(i t))) =
1/(2pi) integral_0^(2pi) (dif t)/(1 - x (1 + o(t))) >=
1/(2pi) integral_0^(2pi) (dif t)/(1 - x (1 - c t)).
$
Этот интеграл считается.
$
1/(2pi) integral_0^(2pi) (dif t)/(1 - x (1 - c t)) =
lr(ln(1 - x + c t x)/(2pi c x) |)_(t = 0)^(t = 2pi) =
1/(2pi c x) ln (1 - x + 2pi c x)/(1 - x) -->_(x -> 1-) +oo.
$
Значит $sum_(n = -oo)^oo P(S_n = 0) = +oo$. Значит каждая точка возвратна.
]
#th(name: "Пойя о возвращении")[
Теперь рассмотрим случайное блуждание в $ZZ^d$, где вероятность попасть в соседнюю точку $1/(2d)$. То есть мы ходим по сетке, и равновероятно выбираем соседа, в которого мы переходим.
Такое случайное блуждание возвратно при $d = 1$ и $d = 2$. Иными словами, если у нас есть пьяный мужик, то он выйдя из дома домой наверняка вернется, а если пьяная ворона --- то уже не факт.
]
#proof[
При $d = 2$ повернем оси на 45#sym.degree. Рассмотрим $xi_n = (xi'_n, xi''_n)$ --- шаги в исходных координатах, и $eta_n = (eta'_n, eta''_n)$ --- в новых. Тогда $P(eta'_n = 1) = 1/2 = P(eta'_n = -1)$, и $P(eta''_n = 1) = 1/2 = P(eta''_n = -1)$. То есть теперь обе проекции смещаются, причем независимо. Тогда это просто 2 параллельных случайных блуждания по одномерной прямой. Формально,
$
P(S_(2n) = 0) = P(tilde(S)_(2n) = 0) = P(S'_(2n) = 0, S''_(2n) = 0) = P(S'_(2n) = 0) P(S''_(2n) = 0) newline(=) (C_(2n)^n dot 1/4^n)^2 sim (1/sqrt(pi n))^2 = 1/(pi n).
$
Ряд расходится.
Понятно, что при $d > 3$ можно следить только за какими-то тремя координатами. Мы не всегда будем по ним ходить, ну и ладно, это возвратимость не улучшит. Поэтому достаточно доказать невозвратность при $d = 3$. Берем ноги в руки и пишем вероятность:
$
p_(0,0) (2n) = sum_(k + j <= n) binom(2n, k, k, j, j, n - k - j, n - k - j) dot 1/(6^(2n)) = С^n_(2n)/6^(2n) sum_(k + j <= n) binom(n, k, j, n - k - j)^2,
$
так как
$
binom(2n, k, k, j, j, n - k - j, n - k - j) = (2n!)/((k!)^2 (j!)^2 ((n - k - j)!)^2) = binom(n, k, j, n - k - j)^2 C_(2n)^n.
$
Причем мы знаем из комбинаторики,
$
sum_(k + j <= n) binom(n, k, j, n - k - j) = 3^n.
$
Надо только разобраться с квадратами. Оценим максимумом:
$
С^n_(2n)/6^(2n) sum_(k + j <= n) binom(n, k, j, n - k - j)^2 <=
C_(2n)^n/(6^(2n)) dot 3^n dot max_(k + j <= n) binom(n, k, j, n - k - j) approx (C^n_(2n))/(12^n) binom(n, n/3, n/3, n/3)
$
и применим Стирлинга:
$
(C^n_(2n))/(12^n) binom(n, n/3, n/3, n/3) sim 1/12^n dot 4^n/sqrt(pi n) dot (sqrt(2 pi n) (n/e)^n)/((sqrt(2 pi n/3)(n/(3e))^(n/3))^3) = 1/cancel(3^n) dot 1/sqrt(pi n) dot 3^(cancel(n) + 3/2)/(2 pi n) = C/(n^(3/2)).
$
Ряд с такими коэффициентами сходится, а значит состояние невозвратно по критерию#rf("recurrent-criterion").
Доказательство того, что если $binom(n, k_0, j_0, n - k_0 - j_0) = max$, то
$
n - k_0 - 1 &<= 2 j_0 &<= n - k_0 + 1 \
n - j_0 - 1 &<= 2 k_0 &<= n - j_0 + 1 \
$
остается читателю.
]
|
|
https://github.com/SWATEngineering/Docs | https://raw.githubusercontent.com/SWATEngineering/Docs/main/src/3_PB/VerbaliInterni/VerbaleInterno_240322/meta.typ | typst | MIT License | #let data_incontro = "22-03-2024"
#let inizio_incontro = "08:00"
#let fine_incontro = "10:00"
#let luogo_incontro = "Discord" |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.